branch_name
stringclasses 15
values | target
stringlengths 26
10.3M
| directory_id
stringlengths 40
40
| languages
sequencelengths 1
9
| num_files
int64 1
1.47k
| repo_language
stringclasses 34
values | repo_name
stringlengths 6
91
| revision_id
stringlengths 40
40
| snapshot_id
stringlengths 40
40
| input
stringclasses 1
value |
---|---|---|---|---|---|---|---|---|---|
refs/heads/master | <repo_name>romanomatthew23/Kuka-Youbot-ECE-470<file_sep>/youbot_ros_hello_world.cpp
//
// Simple demo program that calls the youBot ROS wrapper
//
#include <math.h>
#include <dirent.h>
#include <cstdlib>
#include <iostream>
#include "ros/ros.h"
#include <string>
#include "boost/units/systems/si.hpp"
#include "boost/units/io.hpp"
#include "brics_actuator/JointPositions.h"
#include "geometry_msgs/Twist.h"
ros::Publisher platformPublisher;
ros::Publisher armPublisher;
ros::Publisher gripperPublisher;
void get_block_locations();
#include <unistd.h>
#include <termios.h>
#define PI 3.1415926
double x,y,z;
double z1, y2, z3;
double new_joints[5];
double jnt_0,jnt_1,jnt_2,jnt_3,jnt_4;
char getch() {
char buf = 0;
struct termios old = {0};
if (tcgetattr(0, &old) < 0)
perror("tcsetattr()");
old.c_lflag &= ~ICANON;
old.c_lflag &= ~ECHO;
old.c_cc[VMIN] = 1;
old.c_cc[VTIME] = 0;
if (tcsetattr(0, TCSANOW, &old) < 0)
perror("tcsetattr ICANON");
if (read(0, &buf, 1) < 0)
perror ("read()");
old.c_lflag |= ICANON;
old.c_lflag |= ECHO;
if (tcsetattr(0, TCSADRAIN, &old) < 0)
perror ("tcsetattr ~ICANON");
return (buf);
}
#define TX_MIN -222.0
#define TX_SIZE -161.0
#define TY_MIN 75.0
#define TY_SIZE - 156.0
#define TZ_PERCH 112
#define TZ_GRAB 93
#define TPITCH -2.25
// create a brics actuator message with the given joint position values
brics_actuator::JointPositions createArmPositionCommand(std::vector<double>& newPositions) {
int numberOfJoints = 5;
brics_actuator::JointPositions msg;
if (newPositions.size() < numberOfJoints)
return msg; // return empty message if not enough values provided
for (int i = 0; i < numberOfJoints; i++) {
// Set all values for one joint, i.e. time, name, value and unit
brics_actuator::JointValue joint;
joint.timeStamp = ros::Time::now();
joint.value = newPositions[i];
joint.unit = boost::units::to_string(boost::units::si::radian);
// create joint names: "arm_joint_1" to "arm_joint_5" (for 5 DoF)
std::stringstream jointName;
jointName << "arm_joint_" << (i + 1);
joint.joint_uri = jointName.str();
// add joint to message
msg.positions.push_back(joint);
}
return msg;
}
// create a brics actuator message for the gripper using the same position for both fingers
brics_actuator::JointPositions createGripperPositionCommand(double newPosition) {
brics_actuator::JointPositions msg;
brics_actuator::JointValue joint;
joint.timeStamp = ros::Time::now();
joint.unit = boost::units::to_string(boost::units::si::meter); // = "m"
joint.value = newPosition;
joint.joint_uri = "gripper_finger_joint_l";
msg.positions.push_back(joint);
joint.joint_uri = "gripper_finger_joint_r";
msg.positions.push_back(joint);
return msg;
}
// move platform a little bit back- and forward and to the left and right
void movePlatform() {
geometry_msgs::Twist twist;
// forward
twist.linear.x = 0.05; // with 0.05 m per sec
twist.linear.y = 0.05;
platformPublisher.publish(twist);
ros::Duration(2).sleep();
// backward
twist.linear.x = -0.05;
twist.linear.y = -0.05;
platformPublisher.publish(twist);
ros::Duration(2).sleep();
// to the left
twist.linear.x = 0;
twist.linear.y = 0.05;
platformPublisher.publish(twist);
ros::Duration(2).sleep();
// to the right
twist.linear.y = -0.05;
platformPublisher.publish(twist);
ros::Duration(2).sleep();
// stop
twist.linear.y = 0;
platformPublisher.publish(twist);
}
double float_modulus(double value, double mod) {
while (value < 0) value += mod;
while (value >= mod) value -=mod;
return value;
}
// move arm once up and down
void moveArm(double joint_0, double joint_1, double joint_2, double joint_3, double joint_4) {
//void moveArm() {
brics_actuator::JointPositions msg;
std::vector<double> jointvalues(5);
joint_0 *= -1.0;
joint_1 *= -1.0;
joint_2 *= -1.0;
joint_3 *= -1.0;
joint_4 *= -1.0;
joint_0 += 2.95;
joint_1 += 1.05 + 3.1415/2.0 - .003;
joint_2 += -2.44;
joint_3 += 1.73;
joint_4 += 2.95;
joint_4 = float_modulus(joint_4,2*PI);
joint_0 = float_modulus(joint_0,2*PI);
//Doubling the moves to see if it works
// move arm straight up. values were determined empirically
jointvalues[0] = joint_0;
jointvalues[1] = joint_1;
jointvalues[2] = joint_2;
jointvalues[3] = joint_3;
jointvalues[4] = joint_4;
msg = createArmPositionCommand(jointvalues);
armPublisher.publish(msg);
/*
// move arm back close to calibration position
jointvalues[0] = 0.11;
jointvalues[1] = 0.11;
jointvalues[2] = -0.11;
jointvalues[3] = 0.11;
jointvalues[4] = 0.111;
msg = createArmPositionCommand(jointvalues);
armPublisher.publish(msg);
ros::Duration(2).sleep();
*/
}
// open and close gripper
void moveGripper(int close) {
brics_actuator::JointPositions msg;
if(close) {
// close gripper
msg = createGripperPositionCommand(0);
gripperPublisher.publish(msg);
}
else {
// open gripper
msg = createGripperPositionCommand(0.011);
gripperPublisher.publish(msg);
}
//ros::Duration(3).sleep();
}
bool equ(double a, double b) {
return abs((a-b)/a) < 0.01;
}
#define d5 217.6
#define a1 33.0
#define d1 147.0
#define a2 155.0
#define a3 135.0
#define TRAV_SPEED 1.0
/*void inverse_kin(double * arr, double x,double y,double z,double y2,double z3) {
double * new_joints = new double(5);
for (int i = 0; i < 5; i++)
arr[i] = 0;
arr[0] = atan2(y,x);
z1 = arr[0];
double o3x = x - d5*cos(z1)*sin(y2);
double o3y = y - d5*sin(z1)*sin(y2);
double o3z = z - d5*cos(y2);
printf("o3: %lf %lf %lf\n",o3x,o3y,o3z);
//double r = sqrt((o3x - a1*cos(z1))*(o3x - a1*cos(z1)) + (o3y - a1*sin(z1))*(o3y - a1*sin(z1)));
double r = sqrt(o3x*o3x + o3y*o3y) - a1;
double s = o3z - d1;
double cosq3 = (r*r+s*s - a2*a2 - a3*a3)/(2*a2*a3);
//case 1
arr[4] = z3 + PI;
arr[2] = atan2(-1.0*sqrt(1 - cosq3*cosq3),cosq3);
arr[1] = atan2(s,r) - atan2(a3*sin(arr[2]),a2+a3*cos(arr[2]));
arr[3] = PI/2.0 - y2 - arr[1] - arr[2];
}
*/
void inverse_kin(double * arr, double x,double y,double z,double y2,double z3) {
double * new_joints = new double(5);
for (int i = 0; i < 5; i++)
arr[i] = 0;
arr[0] = atan2(0.0-y,0.0-x);
z1 = arr[0];
double o3x = x - d5*cos(z1)*sin(y2);
double o3y = y - d5*sin(z1)*sin(y2);
double o3z = z - d5*cos(y2);
printf("o3: %lf %lf %lf\n",o3x,o3y,o3z);
//double r = sqrt((o3x - a1*cos(z1))*(o3x - a1*cos(z1)) + (o3y - a1*sin(z1))*(o3y - a1*sin(z1)));
double elbow_rad = sqrt(o3x*o3x + o3y*o3y);
if (o3x*x < 0.0) elbow_rad *= -1;
double r = -elbow_rad - a1;
double s = o3z - d1;
double cosq3 = (r*r+s*s - a2*a2 - a3*a3)/(2*a2*a3);
//case 1
arr[4] = z3 + PI;
arr[2] = atan2(sqrt(1 - cosq3*cosq3),cosq3);
arr[1] = atan2(s,r) - atan2(a3*sin(arr[2]),a2+a3*cos(arr[2]));
arr[3] = PI/2.0 - y2 - arr[1] - arr[2];
}
int main(int argc, char **argv) {
ros::init(argc, argv, "youbot_ros_hello_world");
ros::NodeHandle n;
platformPublisher = n.advertise<geometry_msgs::Twist>("cmd_vel", 1);
armPublisher = n.advertise<brics_actuator::JointPositions>("arm_1/arm_controller/position_command", 1);
gripperPublisher = n.advertise<brics_actuator::JointPositions>("arm_1/gripper_controller/position_command", 1);
sleep(1);
//movePlatform();
//moveArm();
//moveGripper();
//I am going to add in a command terminal interface that controls the robot with the predefined commands
jnt_0 = jnt_1 = jnt_2 = jnt_3 = jnt_4 = 0.0;
int play, grip;
double MOVE_VAL = .01;
double ROT_SPD = .05;
//movePlatform();
moveArm(jnt_0,jnt_1,jnt_2,jnt_3,jnt_4);
int cont = 1;
z1 = y2 = z3 = 0.0;
y2 = 0.0;
x = 0.0;
y = 0.0;
z = 550.0;
while(cont == 1) {
char comm = getch();
printf("%c\n",comm);
switch (comm) {
case '1':
jnt_0 += MOVE_VAL;
break;
case '2':
jnt_0 -= MOVE_VAL;
break;
case '3':
jnt_1 += MOVE_VAL;
break;
case '4':
jnt_1 -= MOVE_VAL;
break;
case '5':
jnt_2 += MOVE_VAL;
break;
case '6':
jnt_2 -= MOVE_VAL;
break;
case '7':
jnt_3 += MOVE_VAL;
break;
case '8':
jnt_3 -= MOVE_VAL;
break;
case '9':
jnt_4 += MOVE_VAL;
break;
case '0':
jnt_4 -= MOVE_VAL;
break;
case 'c':
moveGripper(1);
break;
case 'o':
moveGripper(0);
break;
case 'r':
jnt_0 = jnt_1 = jnt_2 = jnt_3 = jnt_4 = 0.0;
break;
case 'u':
jnt_0 = jnt_1 = jnt_2 = jnt_3 = jnt_4 = 0.0;
jnt_1 = 3.14/2;
break;
case 'p':
printf("claw coordinates:\n");
printf("X =");
scanf("%lf",&x);
printf("Y =");
scanf("%lf",&y);
printf("Z =");
scanf("%lf",&z);
printf("ZYZ Euler Angles:\n");
printf("Z1 determined by claw placement;\n");
printf("Y2 =");
scanf("%lf",&y2);
printf("Z3 =");
scanf("%lf",&z3);
inverse_kin(new_joints,x,y,z,y2,z3);
for (int q = 0; q < 5; q++)
printf("joint[%d] = %lf\n",q,new_joints[q]);
jnt_0 = new_joints[0];
jnt_1 = new_joints[1];
jnt_2 = new_joints[2];
jnt_3 = new_joints[3];
jnt_4 = new_joints[4];
break;
case 'w':
x += TRAV_SPEED;
inverse_kin(new_joints,x,y,z,y2,z3);
jnt_0 = new_joints[0];
jnt_1 = new_joints[1];
jnt_2 = new_joints[2];
jnt_3 = new_joints[3];
jnt_4 = new_joints[4];
break;
case 'a':
y += TRAV_SPEED;
inverse_kin(new_joints,x,y,z,y2,z3);
jnt_0 = new_joints[0];
jnt_1 = new_joints[1];
jnt_2 = new_joints[2];
jnt_3 = new_joints[3];
jnt_4 = new_joints[4];
break;
case 's':
x -= TRAV_SPEED;
inverse_kin(new_joints,x,y,z,y2,z3);
jnt_0 = new_joints[0];
jnt_1 = new_joints[1];
jnt_2 = new_joints[2];
jnt_3 = new_joints[3];
jnt_4 = new_joints[4];
break;
case 'd':
y -= TRAV_SPEED;
inverse_kin(new_joints,x,y,z,y2,z3);
jnt_0 = new_joints[0];
jnt_1 = new_joints[1];
jnt_2 = new_joints[2];
jnt_3 = new_joints[3];
jnt_4 = new_joints[4];
break;
case 'z':
z += TRAV_SPEED;
inverse_kin(new_joints,x,y,z,y2,z3);
jnt_0 = new_joints[0];
jnt_1 = new_joints[1];
jnt_2 = new_joints[2];
jnt_3 = new_joints[3];
jnt_4 = new_joints[4];
break;
case 'x':
z -= TRAV_SPEED;
inverse_kin(new_joints,x,y,z,y2,z3);
jnt_0 = new_joints[0];
jnt_1 = new_joints[1];
jnt_2 = new_joints[2];
jnt_3 = new_joints[3];
jnt_4 = new_joints[4];
break;
case 'n':
y2 += ROT_SPD;
inverse_kin(new_joints,x,y,z,y2,z3);
jnt_0 = new_joints[0];
jnt_1 = new_joints[1];
jnt_2 = new_joints[2];
jnt_3 = new_joints[3];
jnt_4 = new_joints[4];
break;
case 'm':
y2 -= ROT_SPD;
inverse_kin(new_joints,x,y,z,y2,z3);
jnt_0 = new_joints[0];
jnt_1 = new_joints[1];
jnt_2 = new_joints[2];
jnt_3 = new_joints[3];
jnt_4 = new_joints[4];
break;
case 'k':
//k for kamera
get_block_locations();
break;
case 'l':
//list params
printf("X = %lf\n",x);
printf("Y = %lf\n",y);
printf("Z = %lf\n",z);
printf("Z1 = %lf\n",z1);
printf("Y2 = %lf\n",y2);
printf("Z3 = %lf\n",z3);
printf("J1 = %lf\n",jnt_0);
printf("J2 = %lf\n",jnt_1);
printf("J3 = %lf\n",jnt_2);
printf("J4 = %lf\n",jnt_3);
printf("J5 = %lf\n",jnt_4);
break;
case '\n':
break;
case 'q':
default:
std::cout << "exitting";
cont = 0;
}
moveArm(jnt_0,jnt_1,jnt_2,jnt_3,jnt_4);
/*printf("Want to Play? Enter 1: ");
scanf("%d",&play);
if(play) {
printf("Hello. Type in commands to control your fancy Kuka Robot.\nJoint_0 =");
scanf("%lf",&jnt_0);
printf("Joint_1 =");
scanf("%lf",&jnt_1);
printf("Joint_2 =");
scanf("%lf",&jnt_2);
printf("Joint_3 =");
scanf("%lf",&jnt_3);
printf("Joint_4 =");
scanf("%lf",&jnt_4);
printf("Gripper (1=closed) =");
scanf("%d",&grip);
moveArm(jnt_0,jnt_1,jnt_2,jnt_3,jnt_4);
moveGripper(grip);
}
else {
break;
}*/
}
sleep(1);
ros::shutdown();
return 0;
}
void get_block_locations()
{
system("rm -rf /home/youbot/libfreenect-inst2/libfreenect/libfreenect/build/bin/dataloc");
system("timeout 2 /home/youbot/libfreenect-inst2/libfreenect/libfreenect/build/bin/fakenect-record /home/youbot/libfreenect-inst2/libfreenect/libfreenect/build/bin/dataloc > /dev/null");
DIR *dir;
struct dirent *ent;
std::string filename;
if ((dir = opendir ("/home/youbot/libfreenect-inst2/libfreenect/libfreenect/build/bin/dataloc")) != NULL) {
/* print all the files and directories within directory */
while ((ent = readdir (dir)) != NULL) {
if (strstr(ent->d_name,"r")) {
filename = ent->d_name;
break;
}
}
closedir (dir);
} else {
/* could not open directory */
perror ("");
}
std::cout << filename << std::endl;
std::string path = "/home/youbot/libfreenect-inst2/libfreenect/libfreenect/build/bin/dataloc/";
std::string filename_path = path.append(filename); //string of filename included with path
std::string command = "convert ";
std::string output_str = " /home/youbot/libfreenect-inst2/libfreenect/libfreenect/build/bin/dataloc/output.png";
command = (command.append(filename_path).append(output_str));
const char * c = command.c_str();
system(c);
//execute vision
system("rm -f /home/youbot/cv_util/coords.txt");
system("python /home/youbot/cv_util/circles.py > /home/youbot/cv_util/coords.txt");
FILE * fp;
char * line = NULL;
size_t len = 0;
ssize_t read;
fp = fopen("/home/youbot/cv_util/coords.txt", "r");
if (fp == NULL) {
std::cout << "CV Read Error!"<< std::endl;
return;
}
int num_lin = 0;
double coords[2];
while ((read = getline(&line, &len, fp)) != -1) {
printf("Retrieved line of length %zu :\n", read);
coords[num_lin] = std::atoi(line);
printf("%lf\n", coords[num_lin]);
num_lin++;
}
if (num_lin < 2) {
std::cout << "CV Detection Error!" << std::endl;
return;
}
double x_pos = coords[0]/1000000.0;
double y_pos = coords[1]/1000000.0;
double x_dest = TX_MIN + x_pos*TX_SIZE;
double y_dest = TY_MIN + y_pos*TY_SIZE;
x_dest -= 8.0*(1.0-x_pos);
jnt_0 = jnt_1 = jnt_2 = jnt_3 = jnt_4 = 0.0;
jnt_1 = 3.14/2;
moveArm(jnt_0,jnt_1,jnt_2,jnt_3,jnt_4);
sleep(5);
moveGripper(0);
sleep(3);
inverse_kin(new_joints,x_dest,y_dest,TZ_PERCH,TPITCH,0.0);
jnt_0 = new_joints[0];
jnt_1 = new_joints[1];
jnt_2 = new_joints[2];
jnt_3 = new_joints[3];
jnt_4 = new_joints[4];
moveArm(jnt_0,jnt_1,jnt_2,jnt_3,jnt_4);
sleep(5);
inverse_kin(new_joints,x_dest,y_dest,TZ_GRAB-(1.0-x_pos)*7,TPITCH,0.0);
jnt_0 = new_joints[0];
jnt_1 = new_joints[1];
jnt_2 = new_joints[2];
jnt_3 = new_joints[3];
jnt_4 = new_joints[4];
moveArm(jnt_0,jnt_1,jnt_2,jnt_3,jnt_4);
sleep(5);
moveGripper(1);
sleep(3);
jnt_0 = jnt_1 = jnt_2 = jnt_3 = jnt_4 = 0.0;
jnt_1 = 3.14/2;
moveArm(jnt_0,jnt_1,jnt_2,jnt_3,jnt_4);
sleep(5);
}
<file_sep>/README.md
# Kuka-Youbot-ECE-470
<file_sep>/circles.py
#!/usr/bin/python
'''
This example illustrates how to use cv2.HoughCircles() function.
Usage: ./houghcircles.py [<image_name>]
image argument defaults to ../data/board.jpg
'''
import cv2.cv as cv
import cv2
import numpy as np
import sys
def is_appropriate_size(cnt,scale):
return cv2.contourArea(cnt)*scale > .15 and cv2.contourArea(cnt)*scale < .35
def angle_cos(p0, p1, p2):
d1, d2 = (p0-p1).astype('float'), (p2-p1).astype('float')
return abs( np.dot(d1, d2) / np.sqrt( np.dot(d1, d1)*np.dot(d2, d2) ) )
def find_squares(img2,scale):
img = cv2.GaussianBlur(img2, (5, 5), 0)
squares = []
for gray in cv2.split(img):
for thrs in xrange(0, 255, 26):
if thrs == 0:
bin = cv2.Canny(gray, 0, 50, apertureSize=5)
bin = cv2.dilate(bin, None)
else:
retval, bin = cv2.threshold(gray, thrs, 255, cv2.THRESH_BINARY)
contours, hierarchy = cv2.findContours(bin, cv2.RETR_LIST, cv2.CHAIN_APPROX_SIMPLE)
for cnt in contours:
cnt_len = cv2.arcLength(cnt, True)
cnt = cv2.approxPolyDP(cnt, 0.02*cnt_len, True)
if len(cnt) == 4 and is_appropriate_size(cnt,scale) and cv2.isContourConvex(cnt):
cnt = cnt.reshape(-1, 2)
max_cos = np.max([angle_cos( cnt[i], cnt[(i+1) % 4], cnt[(i+2) % 4] ) for i in xrange(4)])
if max_cos < 0.1:
squares.append(cnt)
#print squares
return squares
#print __doc__
try:
fn = sys.argv[1]
except:
fn = "/home/youbot/libfreenect-inst2/libfreenect/libfreenect/build/bin/dataloc/output.png"
src = cv2.imread(fn, 1)
img = cv2.cvtColor(src, cv2.COLOR_BGR2GRAY)
img = cv2.medianBlur(img, 5)
cimg = src.copy() # numpy function
circles = cv2.HoughCircles(img, cv.CV_HOUGH_GRADIENT, 1, 10, np.array([]), 100, 30, 1, 30)
a, b, c = circles.shape
#verify location of square
if b != 4:
sys.exit();
# find center
X_C = 0
Y_C = 0
for i in range(b):
X_C += circles[0][i][0]
Y_C += circles[0][i][1]
X_C /= 4.0;
Y_C /= 4.0;
for i in range(b):
circles[0][i][0] -= X_C;
circles[0][i][1] -= Y_C;
#rotation
ROT = 0
for i in range(b):
ROT += (np.arctan2(circles[0][i][1],circles[0][i][0])) % np.pi/2
ROT /= 4.0
#print(ROT)
ROT -= np.pi/4
ROT *= -1
#print(ROT)
for i in range(b):
circles[0][i][0],circles[0][i][1] = (np.cos(ROT)*circles[0][i][0] - np.sin(ROT)*circles[0][i][1],np.sin(ROT)*circles[0][i][0] + np.cos(ROT)*circles[0][i][1]);
#scaling
X_SC = 0
Y_SC = 0
for i in range(b):
X_SC += np.abs(circles[0][i][0])
Y_SC += np.abs(circles[0][i][1])
X_SC /= 4.0
Y_SC /= 4.0
X_SC = 1/X_SC
Y_SC = 1/Y_SC
for i in range(b):
circles[0][i][0] *= X_SC
circles[0][i][1] *= Y_SC
squares = find_squares(cv2.imread(fn),X_SC*Y_SC)
if not len(squares):
sys.exit();
blk = squares[0];
X_BC = 0
Y_BC = 0
for i in range(4):
X_BC += blk[i][0]
Y_BC += blk[i][1]
X_BC /= 4.0;
Y_BC /= 4.0;
#print (X_BC,Y_BC)
(X_BC_OLD, Y_BC_OLD) = (X_BC,Y_BC)
X_BC -= X_C;
Y_BC -= Y_C;
X_BC,Y_BC = (np.cos(ROT)*X_BC - np.sin(ROT)*Y_BC,np.sin(ROT)*X_BC + np.cos(ROT)*Y_BC);
X_BC *= X_SC
Y_BC *= Y_SC
X_BC = (X_BC + 1.0)/2.0;
Y_BC = (Y_BC + 1.0)/2.0;
print int(1000000*Y_BC)
print int(1000000*X_BC)
#print ""
#for i in range(b):
#print circles[0][i][0],circles[0][i][1]
cv2.drawContours(cimg, [blk], -1, (0, 255, 0), 3 )
cv2.circle(cimg, (int(X_C), int(Y_C)), 2, (0, 255, 0), 3, cv2.CV_AA) # draw
cv2.circle(cimg, (int(X_BC_OLD), int(Y_BC_OLD)), 2, (255, 0, 0), 3, cv2.CV_AA) # draw
#cv2.imshow("source", src)
#cv2.imshow("detected circles", cimg)
cv2.waitKey(0)
| 195914879c9276918334c4963cb7e32621eeb470 | [
"Markdown",
"Python",
"C++"
] | 3 | C++ | romanomatthew23/Kuka-Youbot-ECE-470 | 354c1d6a51ceb360d0416d6edad21e0b5f2c15e5 | 6b238fa6909b1f707d6b3e7acc88c6a9c4951eb4 | |
refs/heads/master | <repo_name>shxx/dva-reactnative-starter<file_sep>/RnDva/src/components/common/CustomNavigationBar.js
import React from 'react';
import { View, Image, TouchableOpacity, Text } from 'react-native';
import { Dim } from '../../utils/config';
import { Actions } from 'react-native-router-flux';
const CustomNavigationBar = ({ navigationBarColor, backImageUri, title, titleColor = '#fff', onRightPress, rightImageUri, rightText }) => {
return (
<View>
<View style={[ styles.statusBarStyle, { backgroundColor: navigationBarColor } ]} />
<View style={[ styles.navigationBarStyle, { backgroundColor: navigationBarColor } ]}>
<TouchableOpacity onPress={Actions.pop} style={(onRightPress && rightText) ? styles.backContainerStyle : ''}>
<Image style={styles.backButtonStyle}
source={{ uri: backImageUri }} />
</TouchableOpacity>
<Text ellipsizeMode='tail' numberOfLines={1} style={[
styles.titleStyle,
{ color: titleColor }
]}>{title}</Text>
{
onRightPress ? <TouchableOpacity onPress={onRightPress}>
{
rightText ? <Text style={[ styles.rightTextStyle, { color: titleColor } ]}
ellipsizeMode='tail' numberOfLines={1}>
{rightText}
</Text> : <Image style={styles.rightImageStyle} source={{ uri: rightImageUri }} />
}
</TouchableOpacity> : <View style={styles.rightImageStyle} />
}
</View>
</View>
);
};
const styles = {
statusBarStyle: {
height: 20*Dim.Factor
},
navigationBarStyle: {
width: Dim.Width,
height: 44*Dim.Factor,
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'flex-start'
},
backButtonStyle: {
width: 20*Dim.Factor,
height: 20*Dim.Factor,
marginLeft: 7*Dim.Factor
},
titleStyle: {
flex: 1,
textAlign: 'center',
fontSize: 18*Dim.Factor,
color: '#fff'
},
rightImageStyle: {
width: 20*Dim.Factor,
height: 20*Dim.Factor,
marginRight: 7*Dim.Factor
},
rightTextStyle: {
width: 80*Dim.Factor,
textAlign: 'right',
fontSize: 16*Dim.Factor,
color: '#fff',
paddingRight: 7*Dim.Factor
},
backContainerStyle: {
width: 80*Dim.Factor,
justifyContent: 'center',
alignItems: 'flex-start'
}
};
export { CustomNavigationBar };
<file_sep>/RnDva/src/components/common/LoginButton.js
import React from 'react';
import { Text, TouchableOpacity } from 'react-native';
import { Dim } from '../../utils/config';
const LoginButton = ({ onPress, title, enable = true, customStyles }) => {
return (
<TouchableOpacity
onPress={onPress}
style={[ styles.buttonStyle, customStyles ]}
disabled={!enable}
>
<Text style={{
fontSize: 18*Dim.Factor,
color: enable ? '#fff' : 'rgba(255,255,255,0.5)'
}}>
{title}
</Text>
</TouchableOpacity>
);
};
const styles = {
buttonStyle: {
width: Dim.Width-44*Dim.Factor,
height: 44*Dim.Factor,
alignItems: 'center',
justifyContent: 'center',
backgroundColor: '#e54748',
borderRadius: 4*Dim.Factor
}
};
export { LoginButton };
<file_sep>/RnDva/src/routes/Register2.js
import React from 'react';
import { StyleSheet, View, Text, Button, TextInput, TouchableOpacity, Image, StatusBar, ScrollView } from 'react-native';
import { connect } from 'dva/mobile';
import { Actions } from 'react-native-router-flux';
import { Dim } from '../utils/config';
import { LoginButton, CustomNavigationBar } from '../components/common';
import { lineIcoStyle, lineDividerStyle, inputStyle, inputTitleStyle } from '../utils/defaults';
class Register2 extends React.Component {
constructor(props, context) {
super(props, context);
this.state = {
captcha: '',
referrer: '',
enable: false
};
}
handleCaptcha(text) {
this.setState({
captcha: text,
enable: text.length > 0 ? true : false
})
}
handleReferrer(text) {
this.setState({
referrer: text,
})
}
getCaptcha() {
this.props.dispatch({
type: 'Newbie/getCaptcha'
})
}
onRegister() {
const { captcha, referrer } = this.state;
this.props.dispatch({
type: 'Newbie/onRegister',
payload: {
captcha: captcha,
referrer: referrer
}
});
}
render() {
const { phone, running, countdown } = this.props;
const { captcha, referrer, enable } = this.state;
return (
<View style={styles.container}>
<StatusBar barStyle='default' />
<CustomNavigationBar navigationBarColor='#f9f9f9' backImageUri='back_lightgray' title='输入验证码' titleColor='#090904' />
<View style={styles.container}>
<View>
<View style={{ marginTop: 40*Dim.Factor, width: Dim.Width-44*Dim.Factor }}>
<Text style={{ fontSize: 16*Dim.Factor, color: '#432f2f' }}>已发送验证码至</Text>
</View>
<View style={styles.phoneContainer}>
<Text style={{ fontSize: 26*Dim.Factor, color: '#090404' }}>{phone}</Text>
</View>
</View>
<View>
<View style={[ styles.inputContainer, { marginTop: 93*Dim.Factor } ]}>
<View style={styles.inputRowContainer}>
<Text style={inputTitleStyle}>验证码</Text>
<TextInput
clearButtonMode='while-editing'
style={[ inputStyle, { marginLeft: 20*Dim.Factor } ]}
value={captcha}
placeholder="6位数字"
placeholderTextColor='#b3a4a4'
maxLength={6}
keyboardType='number-pad'
onChangeText={(text) => this.handleCaptcha(text)} />
<View style={styles.splitLine} />
<TouchableOpacity
onPress={() => this.getCaptcha()}
disabled={running}>
<Text style={{ fontSize: 14*Dim.Factor, color: running ? '#766a6a' : '#e54748'}}>
{ running ? `${countdown} 后重新获取` : '点击重新获取' }
</Text>
</TouchableOpacity>
</View>
<View style={[ lineDividerStyle, { backgroundColor: '#ccc', width: Dim.Width-44*Dim.Factor } ]} />
</View>
<View style={[ styles.inputContainer, { marginTop: 10*Dim.Factor } ]}>
<View style={styles.inputRowContainer}>
<Text style={inputTitleStyle}>推荐人</Text>
<TextInput
clearButtonMode='while-editing'
style={[ inputStyle, { marginLeft: 20*Dim.Factor } ]}
value={referrer}
placeholder="选填"
placeholderTextColor='#b3a4a4'
keyboardType='number-pad'
onChangeText={(text) => this.handleReferrer(text)} />
</View>
<View style={[ lineDividerStyle, { backgroundColor: '#ccc', width: Dim.Width-44*Dim.Factor } ]} />
</View>
</View>
<LoginButton onPress={() => this.onRegister()} title='完成' enable={enable} customStyles={{ marginTop: 65*Dim.Factor }} />
</View>
</View>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
flexDirection: 'column',
alignItems: 'center'
},
inputContainer: {
height: 47*Dim.Factor
},
inputRowContainer: {
flexDirection: 'row',
height: 46*Dim.Factor,
alignItems: 'center'
},
phoneContainer: {
marginTop: 20*Dim.Factor,
alignItems: 'center'
},
splitLine: {
width: 1,
height: 20*Dim.Factor,
marginRight: 10*Dim.Factor,
backgroundColor: '#ccc'
}
});
const mapStateToProps = ({ Newbie }) => {
const { phone, running, countdown } = Newbie;
return { phone, running, countdown };
};
export default connect(mapStateToProps)(Register2);
<file_sep>/RnDva/src/Router.js
import React from 'react';
import { connect } from 'dva/mobile';
import { Scene, Router, Actions } from 'react-native-router-flux';
import { StyleSheet, Text, Image, View } from 'react-native';
import Splash from './components/Splash';
import Homepage from './routes/Homepage';
import Find from './routes/Find';
import More from './routes/More';
import Login from './routes/Login';
import Register from './routes/Register';
import Register2 from './routes/Register2';
class TabIcon extends React.PureComponent {
render() {
const { selected, title } = this.props;
var title_zh, ico, ico_selected = undefined;
switch (title) {
case '首页':
ico = require('../assets/homepage.png');
ico_selected = require('../assets/homepage_icon.png');
break;
case '发现':
ico = require('../assets/find.png');
ico_selected = require('../assets/find_icon.png');
break;
case '更多':
ico = require('../assets/more.png');
ico_selected = require('../assets/more_icon.png');
break;
default:
}
return (
<View style={styles.tabIcon}>
<Image style={styles.tabIconIco} source={ selected ? ico : ico_selected } />
<Text style={[ styles.tabIconTitle, { color: selected ? '#e54748' : '#766a6a' } ]}
>
{title}
</Text>
</View>
);
}
}
const RouterComponent = ({ dispatch }) => {
function onLogout() {
dispatch({ type: 'Auth/logout' });
}
return (
<Router>
<Scene
key="splash"
hideNavBar
passProps
splashText="Hello RnDva"
component={Splash}
/>
<Scene key="auth">
<Scene key="login" component={Login} hideNavBar />
</Scene>
<Scene key="tabbar" tabs={true} hideNavBar tabBarStyle={styles.tabBar}>
<Scene
key="homepage"
title="首页"
icon={TabIcon}
initial
>
<Scene key="public" component={Homepage} hideNavBar />
<Scene key="authorized" component={Homepage} hideNavBar />
</Scene>
<Scene key="find" title="发现" component={Find} icon={TabIcon} />
<Scene key="more" title="更多" component={More} icon={TabIcon} />
</Scene>
<Scene key="register" component={Register} hideNavBar />
<Scene key="register2" component={Register2} hideNavBar />
</Router>
);
};
let styles = StyleSheet.create({
tabBar: {
borderTopWidth : 1,
borderColor : '#ccc'
},
tabIcon: {
flex: 1,
justifyContent: 'center',
alignItems: 'center'
},
tabIconIco: {
width: 22,
height: 22
},
tabIconTitle: {
marginTop: 5,
fontSize: 10
},
redNav: {
backgroundColor: '#E44747'
},
redNavTitle: {
color: '#fff'
},
redNavRightButtonText: {
color: '#fff',
fontSize: 14,
marginTop: 3
},
whiteNav: {
backgroundColor: '#fff',
borderBottomColor: '#ddd',
},
whiteNavTitle: {
color: '#090404'
},
});
export default connect()(RouterComponent);
<file_sep>/RnDva/src/utils/config.js
import Dimensions from 'Dimensions';
var debug = true;
var needCheckVersion = false;
var { height, width } = Dimensions.get('window');
module.exports = {
Dim: {
Width: width,
Height: height,
Factor: width/375
}
};
<file_sep>/RnDva/src/routes/Homepage.js
import React, { Component } from 'react';
import { StyleSheet, Text, View, Button } from 'react-native';
import { connect } from 'dva/mobile';
import { Actions } from 'react-native-router-flux';
import { Motion, spring } from 'react-motion';
import { Dim } from '../utils/config';
class HomepageComponent extends Component {
constructor(props, context) {
super(props, context);
this.state = {
open: '',
};
}
handleClick() {
this.setState({
open: !this.state.open
});
}
render() {
return (
<View style={styles.container}>
<Text>This is homepage.</Text>
<Button title="login" onPress={Actions.auth} />
<Button title="register" onPress={Actions.register} />
<Button title="react-motion in rn, pls click it"
onPress={() => this.handleClick()} />
<Motion style={{
x: spring(this.state.open ? Dim.Width-50 : 0),
a: spring(this.state.open ? 1 : 0.2)
}}>
{({x, a}) =>
<View style={{ width: Dim.Width, height: 50, backgroundColor: '#f9f9f9' }}>
<View style={{
width: 50,
height: 50,
position: 'absolute',
left: x,
opacity: a,
backgroundColor: '#ffc2da' }}>
</View>
</View>
}
</Motion>
</View>
);
}
}
let styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center'
},
});
const mapStateToProps = ({ Homepage }) => {
return {};
};
export default connect(mapStateToProps)(HomepageComponent);
<file_sep>/RnDva/src/components/common/index.js
export * from './LoginButton';
export * from './CustomNavigationBar';
<file_sep>/RnDva/src/models/Newbie.js
import { delay } from "../services/Countdown";
const INITIAL_STATE = {
phone: '',
password: '',
secure: true,
enable: false,
checked: true,
running: false,
countdown: 0,
loading: false
};
export default {
namespace: 'Newbie',
state: { ...INITIAL_STATE },
reducers: {
showLoading(state) {
return { ...state, loading: true, error: '' };
},
hideLoading(state) {
return { ...state, loading: false, error: '' };
},
phoneChanged(state, action) {
return { ...state,
phone: action.payload,
enable: (action.payload.length == 11 && state.password.length >= 6) ? true : false
};
},
passwordChanged(state, action) {
return { ...state,
password: action.payload,
enable: (action.payload.length >= 6 && state.phone.length == 11) ? true : false
};
},
secureChanged(state, action) {
return { ...state, secure: !state.secure };
},
checkedChanged(state, action) {
return { ...state, checked: !state.checked };
},
getCaptchaSuccess(state, action) {
return {
...state,
running: true,
countdown: 60
}
},
countdown(state, action) {
return { ...state, countdown: action.payload };
},
stopCountdown(state) {
return { ...state, running: false, countdown: 0 };
},
},
effects: {
*getCaptcha ({
payload
}, {call, put, select}) {
yield put({type: 'showLoading'})
const ret = { data: { result_code: 'SUCCESS' } } // 假定获取验证码成功
if (ret.data.result_code === "SUCCESS") {
yield put({type: 'getCaptchaSuccess'});
yield put({type: 'hideLoading'})
yield delay(1000); // 补1s
while (true) {
let countdown = yield select(state => state.Newbie.countdown);
if (countdown > 0) {
countdown--
yield put({ type: 'countdown', payload: countdown });
yield delay(1000);
} else {
yield put({ type: 'stopCountdown' });
return;
}
}
} else {
yield put({type: 'hideLoading'})
}
},
* onRegister ({
payload
}, {call, put}) {
console.log('onRegister', payload);
}
},
subscriptions: {
}
};
<file_sep>/RnDva/src/components/Login.js
import React from 'react';
import {
View,
Text,
TextInput,
TouchableOpacity,
Image,
StatusBar
} from 'react-native';
import { Actions } from 'react-native-router-flux';
import { Dim } from '../utils/config';
import { LoginButton, CustomNavigationBar } from './common';
import { lineIcoStyle, lineDividerStyle, inputStyle } from '../utils/defaults';
const LoginComponent = ({ phone, password, secure, enable, handlePhone, handlePassword, onSecure, onLogin }) => {
return (
<View style={styles.container}>
<StatusBar barStyle='default' />
<CustomNavigationBar navigationBarColor='transparent' backImageUri='close_lightgray' />
<View style={styles.container}>
<View style={styles.logoContainer}>
<Image style={{
width: 98*Dim.Factor,
height: 98*Dim.Factor
}} source={{ uri: 'logo_ico' }} />
</View>
<View>
<View style={styles.inputContainer}>
<TextInput
style={inputStyle}
clearButtonMode='while-editing'
placeholder="手机号"
placeholderTextColor='#432f2f'
keyboardType='number-pad'
maxLength={11}
value={phone}
onChangeText={(text) => handlePhone(text)} />
<View style={[ lineDividerStyle, { backgroundColor: '#ccc', width: Dim.Width-44*Dim.Factor } ]} />
</View>
<View style={[ styles.inputContainer, { marginTop: 10 } ]}>
<View style={styles.inputRowContainer}>
<TextInput
style={inputStyle}
clearButtonMode='while-editing'
placeholder="登录密码"
placeholderTextColor='#432f2f'
secureTextEntry={secure}
maxLength={16}
value={password}
onChangeText={(text) => handlePassword(text)} />
<TouchableOpacity onPress={onSecure}>
<Image style={lineIcoStyle} source={ secure ? { uri: 'eye_close_lightgray' } : { uri: 'eye_open_red' }} />
</TouchableOpacity>
</View>
<View style={[ lineDividerStyle, { backgroundColor: '#ccc', width: Dim.Width-44*Dim.Factor } ]} />
</View>
</View>
<View style={styles.additionalContainer}>
<TouchableOpacity onPress={Actions.forgot}>
<Text style={{
fontSize: 14*Dim.Factor,
color: '#968d8d'
}}>忘记密码</Text>
</TouchableOpacity>
<TouchableOpacity onPress={Actions.register}>
<Text style={{
fontSize: 14*Dim.Factor,
color: '#e54748'
}}>注册账户</Text>
</TouchableOpacity>
</View>
<LoginButton onPress={onLogin} title='登录' enable={enable} customStyles={{ marginTop: 65*Dim.Factor }} />
</View>
</View>
);
};
const styles = {
container: {
flex: 1,
alignItems: 'center'
},
logoContainer: {
marginTop: 35*Dim.Factor,
marginBottom: 55*Dim.Factor,
},
inputContainer: {
height: 47*Dim.Factor
},
inputRowContainer: {
flexDirection: 'row',
height: 46*Dim.Factor,
alignItems: 'center'
},
additionalContainer: {
flexDirection: 'row',
justifyContent: 'space-between',
alignItems: 'center',
width: Dim.Width-44*Dim.Factor,
marginTop: 20*Dim.Factor
}
};
export default LoginComponent;
<file_sep>/README.md
# dva-reactnative-starter
This project is designed to help those who use dva to develop an native-app(it also can develop web app). In a word, this is a simple example.
[](https://npmjs.org/package/antd-init)
[Dva](https://github.com/dvajs/dva)
## What's include?
1. [Dva](https://github.com/dvajs/dva), Lightweight front-end framework based on redux, redux-saga and [email protected]. (Inspired by elm and choo)
[Dva Knowledgemap](https://github.com/dvajs/dva-knowledgemap)
[Quick Start (12 步 30 分钟,完成用户管理的 CURD 应用) (react+dva+antd)](https://github.com/sorrycc/blog/issues/18)
2. [React-Motion](https://github.com/chenglou/react-motion), A spring that solves your animation problems.
It's Works with React-Native v0.18+. In addition, I have written two examples on the web application. If you are interested in the animation, you should go to look at it. (Additional knowledge.)
[react-motion-tab-animate](https://github.com/yuzhouisme/react-motion-tab-animate)
[react-motion-nav-animate](https://github.com/yuzhouisme/react-motion-nav-animate)
## Screenshot

## Getting started
1. Clone this repo.
2. Modify something in package.json like name to "your-project-name".
3. Run npm install.
```bash
$ npm install
```
4. Run.
```bash
$ react-native run-ios
```
## Explanation
1. To know the directory structure.
```
$ tree . -L 3
.
├── android
├── assets // saved image resources who variable
├── index.android.js // root entrance for android platform!!
├── index.ios.js // root entrance!!
├── ios
├── node_modules
├── package.json
├── src
│ ├── Router.js // all routing
├── components // dumb component
│ ├── Login.js
│ ├── Splash.js
│ └── common
├── models
│ ├── Auth.js
│ ├── Find.js
│ ├── Homepage.js
│ ├── Initial.js
│ └── Newbie.js
├── routes // smart component
│ ├── Find.js
│ ├── Homepage.js
│ ├── Login.js
│ ├── More.js
│ ├── Register.js
│ └── Register2.js
├── services
├── utils // such as config, request, defaults
└── yarn.lock
```
2. The whole entrance is index.jsx. I try to describe clearly why import that.
```
...
const app = dva();
// Load models. In web application can use dynamic routing, But in native-app maybe don't need
app.model(Initial);
app.model(Auth);
app.model(Homepage);
app.model(Newbie);
app.model(Find);
app.router(() => <Router />);
AppRegistry.registerComponent('RnDva', () => app.start());
```
3. Dva/Redux/React-Router, reading some documents will help you.
4. Look at 'subscriptions' (in models/Initial.js), when app in Splash screen, then will jump to Homepage after 3s.
```
...
subscriptions: {
initialize() {
_.delay(() => {
Actions.tabbar({ type: 'reset' });
}, 3000);
}
}
```
## Inspiration
Thanks to [dvajs group](https://github.com/dvajs) for providing the lightweight framework for developers. And in work I am a mobile application developer as android/iOS platform, before using dva, I have been using the reactnative+reudx+redux-thunk. I have subscribed to issue list for a long time. I saw a lot of issues have been proposed, and soon be solved. Thanks to the team and chencheng (云谦) once again.
Thank you for reading.
##
logo_ico.png from [rezzzzzzz's Oh My D.VA](https://dribbble.com/rezzzzzz) who in [dribbble](https://dribbble.com). Thank rezzzzzzz! She's so pink :)
<file_sep>/RnDva/index.ios.js
/**
* Sample React Native App
* https://github.com/facebook/react-native
* @flow
*/
import React from 'react';
import { AppRegistry } from 'react-native';
import dva from 'dva/mobile';
import Router from './src/Router';
import Initial from './src/models/Initial';
import Auth from './src/models/Auth';
import Homepage from './src/models/Homepage';
import Newbie from './src/models/Newbie';
const app = dva();
app.model(Initial);
app.model(Auth);
app.model(Homepage);
app.model(Newbie);
app.router(() => <Router />);
AppRegistry.registerComponent('RnDva', () => app.start());
<file_sep>/RnDva/src/services/Auth.js
export function signInWithPhoneAndPassword(phone, password) {
return { user: {}, err: true };
}
export function signOut() {
return true;
}
<file_sep>/RnDva/src/routes/Register.js
import React from 'react';
import { StyleSheet, View, Text, Button, TextInput, TouchableOpacity, Image, StatusBar, ScrollView } from 'react-native';
import { connect } from 'dva/mobile';
import { Actions } from 'react-native-router-flux';
import { Dim } from '../utils/config';
import { LoginButton, CustomNavigationBar } from '../components/common';
import { lineIcoStyle, lineDividerStyle, inputStyle, inputTitleStyle } from '../utils/defaults';
class Register extends React.Component {
handlePhone(text) {
this.props.dispatch({
type: 'Newbie/phoneChanged',
payload: text
})
}
handlePassword(text) {
this.props.dispatch({
type: 'Newbie/passwordChanged',
payload: text
})
}
onChecked() {
this.props.dispatch({
type: 'Newbie/checkedChanged',
})
}
onSecure() {
this.props.dispatch({
type: 'Newbie/secureChanged',
})
}
render() {
const { phone, password, secure, enable, checked } = this.props;
return (
<View style={styles.container}>
<StatusBar barStyle='default' />
<CustomNavigationBar navigationBarColor='transparent' backImageUri='close_lightgray' />
<View style={styles.container}>
<View style={styles.logoContainer}>
<Image style={{
width: 98*Dim.Factor,
height: 98*Dim.Factor
}} source={{ uri: 'logo_ico' }} />
</View>
<View>
<View style={styles.inputContainer}>
<View style={styles.inputRowContainer}>
<Text style={inputTitleStyle}>
{ "手机号 " }
</Text>
<TextInput
clearButtonMode='while-editing'
style={[ inputStyle, { fontSize: 14*Dim.Factor, marginLeft: 20*Dim.Factor } ]}
value={phone}
keyboardType='number-pad'
maxLength={11}
placeholder="中国大陆11手机号码"
placeholderTextColor='#b3a4a4'
onChangeText={(text) => this.handlePhone(text)}
/>
</View>
<View style={[ lineDividerStyle, { backgroundColor: '#ccc', width: Dim.Width-44*Dim.Factor } ]} />
</View>
<View style={styles.inputContainer}>
<View style={styles.inputRowContainer}>
<Text style={inputTitleStyle}>登录密码</Text>
<TextInput
clearButtonMode='while-editing'
style={[ inputStyle, { fontSize: 14*Dim.Factor, marginLeft: 20*Dim.Factor } ]}
value={password}
secureTextEntry={secure}
maxLength={16}
placeholder="6~16位数字、字母或符号组合"
placeholderTextColor='#b3a4a4'
returnKeyType='done'
onChangeText={(text) => this.handlePassword(text)}
/>
<TouchableOpacity onPress={() => this.onSecure()}>
<Image style={lineIcoStyle} source={ secure ? { uri: 'eye_close_lightgray' } : { uri: 'eye_open_red' }} />
</TouchableOpacity>
</View>
<View style={[ lineDividerStyle, { backgroundColor: '#ccc', width: Dim.Width-44*Dim.Factor } ]} />
</View>
</View>
<LoginButton onPress={Actions.register2} title='下一步' enable={enable} customStyles={{ marginTop: 65*Dim.Factor }} />
<View style={styles.tipsContainer}>
<TouchableOpacity onPress={() => this.onChecked()}>
<Image style={styles.checkImage} source={ checked ? { uri: 'check_colorful_round' } : { uri: 'uncheck_lightgray_round' }} />
</TouchableOpacity>
<Text style={styles.tips}>我已阅读并同意</Text>
<TouchableOpacity>
<Text style={styles.agreement}>《用户服务协议》</Text>
</TouchableOpacity>
</View>
</View>
</View>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
flexDirection: 'column',
alignItems: 'center',
},
logoContainer: {
marginTop: 35*Dim.Factor,
marginBottom: 55*Dim.Factor,
},
inputContainer: {
height: 47*Dim.Factor
},
inputRowContainer: {
flexDirection: 'row',
height: 46*Dim.Factor,
alignItems: 'center'
},
tips: {
fontSize: 14*Dim.Factor,
color: '#968d8d'
},
agreement: {
fontSize: 14*Dim.Factor,
color: '#e54748'
},
tipsContainer: {
flexDirection: 'row',
alignItems: 'center',
marginTop: 15*Dim.Factor,
},
checkImage: {
width: 20*Dim.Factor,
height: 20*Dim.Factor,
marginLeft: 4*Dim.Factor,
marginRight: 2*Dim.Factor
}
});
const mapStateToProps = ({ Newbie }) => {
const { phone, password, secure, enable, checked, loading } = Newbie;
return { phone, password, secure, enable, checked, loading };
};
export default connect(mapStateToProps)(Register);
<file_sep>/RnDva/android/settings.gradle
rootProject.name = 'RnDva'
include ':app'
<file_sep>/RnDva/src/routes/Login.js
import React from 'react';
import { connect } from 'dva/mobile';
import { Actions } from 'react-native-router-flux';
import LoginComponent from '../components/Login';
class Login extends React.Component {
render() {
const { phone, password, secure, enable, dispatch } = this.props;
const loginProps = {
phone,
password,
secure,
enable,
handlePhone(text) {
dispatch({
type: 'Auth/phoneChanged',
payload: text
})
},
handlePassword(text) {
dispatch({
type: 'Auth/passwordChanged',
payload: text
})
},
onSecure() {
console.log('onSecure');
dispatch({
type: 'Auth/secureChanged',
})
},
onLogin() {
console.log('onLogin');
dispatch({
type: 'Auth/login',
});
}
};
return (
<LoginComponent {...loginProps} />
);
}
}
const mapStateToProps = ({ Auth }) => {
const { phone, password, secure, enable, loading } = Auth;
return { phone, password, secure, enable, loading };
};
export default connect(mapStateToProps)(Login);
| fee49bf9570bb3aab3bb62239a61bf4ffd48395b | [
"JavaScript",
"Markdown",
"Gradle"
] | 15 | JavaScript | shxx/dva-reactnative-starter | 6b1b1cdeffb255cbc6e7667ec8a08bea1012e8f4 | edc4ba3b6c7c1cde6dd9ff1222f3329c1dd7828b | |
refs/heads/develop | <repo_name>carrielord/FeedTheNeedAngular<file_sep>/src/app/component/postingdetail/postingdetail.component.ts
import { Component, OnInit } from '@angular/core';
import { PostingService } from '../../services/posting.service';
import { MatTableDataSource } from '@angular/material';
import { Posting } from '../../models/posting';
import { ActivatedRoute } from '@angular/router';
import { User } from '../../models/user';
@Component({
selector: 'app-postingdetail',
templateUrl: './postingdetail.component.html',
styleUrls: ['./postingdetail.component.css']
})
export class PostingdetailComponent implements OnInit {
posting: Posting;
user: User;
constructor(private _postingService: PostingService, private _activatedRoute: ActivatedRoute) { }
columnNames = ['Title:', 'Details:', 'Address:', 'City:', 'State:', 'Organization:', 'Category:', 'Date Posted:', 'Available Until:', 'Is Completed?']
dataSource: MatTableDataSource<Posting>
ngOnInit() {
this._activatedRoute.paramMap.subscribe(routeData => {
this._postingService.getPostDetail(routeData.get('id')).subscribe((singlePost: Posting) => {
this.posting = singlePost;
});
});
}
}
<file_sep>/src/app/component/organization/organization-delete/organization-delete.component.ts
import { Component, OnInit } from '@angular/core';
import { ActivatedRoute, Router } from '@angular/router';
import { Organization } from '../../../models/organization';
import { OrganizationService } from '../../../services/organization.service';
import { User } from 'src/app/models/user';
import { UserService } from 'src/app/services/user.service';
@Component({
selector: 'app-organization-delete',
templateUrl: './organization-delete.component.html',
styleUrls: ['./organization-delete.component.css']
})
export class OrganizationdeleteComponent implements OnInit {
organization: Organization;
user: User;
constructor(private _organizationService: OrganizationService, private _userService: UserService, private _ar: ActivatedRoute, private _router: Router) {
this._ar.paramMap.subscribe(p => {
this._organizationService.getOrganization(p.get('id')).subscribe((singleOrganization: Organization) => {
this.organization = singleOrganization;
});
});
}
onDelete() {
this._organizationService.deleteOrganization(this.organization.OrganizationID).subscribe(() => {
this._router.navigate(['/organizations']);
});
}
ngOnInit() {
this.getUserRole();
}
getUserRole(){
this._userService.getUser().subscribe((singleUser: User) => {
this.user = singleUser;
console.log(this.user.Role)
})
}
isAdmin(){
if(this.user.Role=='Admin'){
return true;
}
}
}
<file_sep>/src/app/component/postinguserdetail/postinguserdetail.component.ts
import { Component, OnInit } from '@angular/core';
import { Posting } from 'src/app/models/posting';
import { PostingService } from 'src/app/services/posting.service';
import { ActivatedRoute, Router } from '@angular/router';
import { User } from '../../models/User';
import { MatTableDataSource } from '@angular/material';
@Component({
selector: 'app-postinguserdetail',
templateUrl: './postinguserdetail.component.html',
styleUrls: ['./postinguserdetail.component.css']
})
export class PostinguserdetailComponent implements OnInit {
posting: Posting;
user: User;
constructor(private _postingService: PostingService, private _activatedRoute: ActivatedRoute, private _router: Router) {
this._activatedRoute.paramMap.subscribe(p => {
this._postingService.getPostDetail(p.get('id')).subscribe((singlePost: Posting) => {
this.posting = singlePost;
this.getUserInfo();
});
});
}
columnNames: ['First Name:', 'Last Name:', 'Phone Number:', 'Email:']
dataSource: MatTableDataSource<User>
ngOnInit() {
}
getUserInfo(){
this._postingService.getPostUserDetail(this.posting.UserID).subscribe((userPostDetails: User) =>{
this.user = userPostDetails;
});
}
}
<file_sep>/src/app/component/organization/organization-edit/organization-edit.component.ts
import { Component, OnInit } from '@angular/core';
import { FormGroup, FormBuilder, FormControl } from '@angular/forms';
import { OrganizationService } from 'src/app/services/organization.service';
import { ActivatedRoute, Router } from '@angular/router';
import { Organization } from 'src/app/models/organization';
import { User } from 'src/app/models/user';
import { UserService } from 'src/app/services/user.service';
@Component({
selector: 'app-notorganizatione-edit',
templateUrl: './organization-edit.component.html',
styleUrls: ['./organization-edit.component.css']
})
export class OrganizationeditComponent implements OnInit {
organization: Organization;
user: User;
editOrganizationForm: FormGroup;
constructor(private _form: FormBuilder,
private _organizationService:OrganizationService,
private _userService: UserService,
private _ar: ActivatedRoute,
private _router: Router) {
this._ar.paramMap.subscribe(p => {
this._organizationService.getOrganization(p.get('id')).subscribe((singleOrganization: Organization) => {
this.organization = singleOrganization;
this.createForm();
});
});
}
ngOnInit() {
this.getUserRole();
}
createForm() {
this.editOrganizationForm = this._form.group({
OrganizationID: new FormControl(this.organization.OrganizationID),
OrganizationName: new FormControl(this.organization.OrganizationName),
OrganizationLink: new FormControl(this.organization.OrganizationLink),
OrganizationBio: new FormControl(this.organization.OrganizationBio)
})
}
getUserRole(){
this._userService.getUser().subscribe((singleUser: User) => {
this.user = singleUser;
console.log(this.user.Role)
})
}
isAdmin(){
if(this.user.Role=='Admin'){
return true;
}
}
onSubmit(form) {
console.log(this.organization)
const updateOrganization: Organization = {
OrganizationID: form.value.OrganizationID,
OrganizationName: form.value.OrganizationName,
OrganizationLink: form.value.OrganizationLink,
OrganizationBio: form.value.OrganizationBio
};
this._organizationService.updateOrganization(updateOrganization).subscribe(d => {
this._router.navigate(['/organizations']);
})
}
}
<file_sep>/src/app/component/userdeleteadmin/userdeleteadmin.component.html
<h1>Delete</h1>
<h4>Are you sure you want to delete this account and all the posts associated with it? This can't be undone</h4>
<hr>
<div *ngIf="isAdmin()">
<div>
<label for="">UserID</label>
<span> {{user.UserID}}</span>
</div>
<div>
<label for="">First Name</label>
<span> {{user.FirstName}}</span>
</div>
<div>
<label for="">Last Name</label>
<span> {{user.LastName}}</span>
</div>
<div>
<label for="">Email</label>
<span> {{user.Email}}</span>
</div>
<div>
<label for="">Phone Number</label>
<span> {{user.PhoneNumber}}</span>
</div>
</div>
<button mat-button color="warn" (click)="onDelete()">Delete</button>
<button mat-button color="primary" routerLink="/userlist">Back to list</button>
<file_sep>/src/app/component/userdetails/userdetails.component.ts
import { Component, OnInit } from '@angular/core';
import{ActivatedRoute} from '@angular/router';
import { User} from '../../models/user';
import { UserService } from '../../services/user.service';
import { AuthService } from 'src/app/services/auth.service';
import { UserRole } from 'src/app/models/userrole';
@Component({
selector: 'app-userdetails',
templateUrl: './userdetails.component.html',
styleUrls: ['./userdetails.component.css']
})
export class UserdetailsComponent implements OnInit {
user: User;
userRole: UserRole;
constructor(private _activatedRoute: ActivatedRoute, private _userService: UserService, private _authService: AuthService) { }
ngOnInit() {
// this._activatedRoute.paramMap.subscribe(routeData=>{this._userService.getUser(routeData.get('id')).subscribe((singleUser: User)=>{
// this.user= singleUser;
// });
// });
this._userService.getUser().subscribe((singleUser: User) => {
this.user = singleUser;
console.log(this.user.Role)
})
}
isAdmin(){
if(this.user.Role=='Admin'){
return true;
}
}
// isItAdmin(){
// this._authService.isAdmin().subscribe((userRole: UserRole) => {
// this.userRole = userRole;
// })
// if(this.userRole.Role=='Admin'){
// return true;
// }
}
// checkAdmin(){
// if (this.user.Email == '<EMAIL>')
// return true;
// }
<file_sep>/src/app/component/personalizedlistofpostings/personalizedlistofpostings.component.ts
import { Component, OnInit } from '@angular/core';
import { PostingService } from '../../services/posting.service';
import { Posting } from '../../models/posting';
import { MatTableDataSource } from '@angular/material';
import { User } from '../../models/user';
@Component({
selector: 'app-personalizedlistofpostings',
templateUrl: './personalizedlistofpostings.component.html',
styleUrls: ['./personalizedlistofpostings.component.css']
})
export class PersonalizedlistofpostingsComponent implements OnInit {
columnNames = ['details', 'Title', 'State', 'Available Until', 'buttons']
dataSource: MatTableDataSource<Posting>
user: User;
posting: Posting;
constructor(private _postingService: PostingService) { }
ngOnInit() {
this._postingService.getPostList().subscribe((postings: Posting[]) => {
this.dataSource = new MatTableDataSource<Posting>(postings);
// if (this.posting.UserID != this.user.UserID){
// }
});
}
}
<file_sep>/src/app/component/postingedit/postingedit.component.ts
import { Component, OnInit } from '@angular/core';
import { FormGroup, FormBuilder, FormControl } from '@angular/forms';
import { PostingService } from '../../services/posting.service';
import { Posting } from '../../models/posting';
import { ActivatedRoute, Router } from '@angular/router';
@Component({
selector: 'app-postingedit',
templateUrl: './postingedit.component.html',
styleUrls: ['./postingedit.component.css']
})
export class PostingeditComponent implements OnInit {
posting: Posting;
postingEditForm: FormGroup;
constructor(private _form: FormBuilder, private _postingService: PostingService, private _activatedRoute: ActivatedRoute, private _router: Router) {
this._activatedRoute.paramMap.subscribe(p => {
this._postingService.getPostDetail(p.get('id')).subscribe((singlePost: Posting) => {
this.posting = singlePost;
console.table(this.posting);
this.createForm();
});
});
}
ngOnInit() {
}
createForm() {
this.postingEditForm = this._form.group({
PostID: new FormControl(this.posting.PostID),
Title: new FormControl(this.posting.Title),
Details: new FormControl(this.posting.Details),
Address: new FormControl(this.posting.Address),
City: new FormControl(this.posting.City),
State: new FormControl(this.posting.State),
NameOfProvider: new FormControl(this.posting.NameOfProvider),
Category: new FormControl(this.posting.Category),
DateAvailable: new FormControl(this.posting.DateAvailable),
IsCompleted: new FormControl(this.posting.IsCompleted)
});
}
onSubmit(form) {
const updatePost: Posting = {
PostID: form.value.PostID,
Title: form.value.Title,
Details: form.value.Details,
Address: form.value.Address,
City: form.value.City,
State: form.value.State,
NameOfProvider: form.value.NameOfProvider,
Category: form.value.Category,
DateAvailable: form.value.DateAvailable,
IsCompleted: form.value.IsCompleted
};
console.log(this.postingEditForm.value);
this._postingService.updatePost(updatePost).subscribe(d => {
this._router.navigate(['/posting/list'])
});
}
}
<file_sep>/src/app/services/organization.service.ts
import { Injectable } from '@angular/core';
import { HttpClient, HttpHeaders } from '@angular/common/http';
import { Organization } from '../models/organization';
import { APIURL } from '../../environments/environment.prod';
// const APIURL = 'https://localhost:44381/api';
@Injectable({
providedIn: 'root'
})
export class OrganizationService {
constructor(private _http: HttpClient ) { }
getOrganization(id: string) {
return this._http.get(`${APIURL}/Organization/${id}`, { headers: this.getHeaders() });
}
getOrganizations() {
return this._http.get(`${APIURL}/Organization`, { headers: this.getHeaders() });
}
createOrganization(organization: Organization) {
return this._http.post(`${APIURL}/Organization`, organization, { headers: this.getHeaders() });
}
private getHeaders() {
return new HttpHeaders().set('Authorization', `Bearer ${localStorage.getItem('id_token')}`)
}
updateOrganization(organization: Organization) {
return this._http.put(`${APIURL}/Organization`, organization, { headers: this.getHeaders() });
}
deleteOrganization(id: number) {
return this._http.delete(`${APIURL}/Organization/${id}`, {headers: this.getHeaders() });
}
}
<file_sep>/src/environments/environment.prod.ts
export const environment = {
production: true
};
export let APIURL = '';
switch (window.location.hostname) {
case 'feedaneed.herokuapp.com':
APIURL = 'https://feedtheneed.azurewebsites.net'
break;
default:
APIURL = 'http://localhost:44381';
}<file_sep>/src/app/component/useredit/useredit.component.ts
import { Component, OnInit } from '@angular/core';
import { FormGroup, FormBuilder, FormControl } from '@angular/forms';
import { UserService } from 'src/app/services/user.service';
import { User } from 'src/app/models/user';
import { Router } from '@angular/router';
@Component({
selector: 'app-useredit',
templateUrl: './useredit.component.html',
styleUrls: ['./useredit.component.css']
})
export class UserEditComponent implements OnInit {
user: User;
editUserForm: FormGroup;
constructor(private _form: FormBuilder,
private _userService:UserService, private _router: Router) {
this._userService.getUser().subscribe((singleUser: User) => {
this.user = singleUser;
this.createForm();
});
}
ngOnInit() {
}
createForm() {
this.editUserForm = this._form.group({
// FirstName: string;
// LastName: string;
// Email: string;
// PhoneNumber: string;
UserID: new FormControl(this.user.UserID),
FirstName: new FormControl(this.user.FirstName),
LastName: new FormControl(this.user.LastName),
Email: new FormControl(this.user.Email),
PhoneNumber: new FormControl(this.user.PhoneNumber)
});
}
onSubmit(form) {
console.log(this.user)
const updateUser: User = {
// FirstName: string;
// LastName: string;
// Email: string;
// PhoneNumber: string;
UserID: form.value.UserID,
FirstName: form.value.FirstName,
LastName: form.value.LastName,
Email: form.value.Email,
PhoneNumber: form.value.PhoneNumber
};
this._userService.updateUser(updateUser).subscribe(d => {this._router.navigate(['/userdetails']);
})
}
}<file_sep>/src/app/services/posting.service.ts
import { Injectable } from '@angular/core';
import{Posting} from '../models/posting';
import { HttpClient, HttpHeaders } from '@angular/common/http';
import { APIURL } from '../../environments/environment.prod';
// const APIURL = 'https://localhost:44381/api';
@Injectable({
providedIn: 'root'
})
export class PostingService {
constructor(private _http: HttpClient) { }
createPost(posting: Posting){
return this._http.post(`${APIURL}/Posting`, posting, { headers: this.getHeaders() });
}
getPostList(){
return this._http.get(`${APIURL}/Posting`, { headers: this.getHeaders() });
}
getPostDetail(id: string){
return this._http.get(`${APIURL}/Posting/${id}`, { headers: this.getHeaders() });
}
getPostUserDetail(id: string){
return this._http.get(`${APIURL}/Account/PostingDetailUser?id=${id}`, {headers: this.getHeaders()})
}
updatePost(posting: Posting){
return this._http.put(`${APIURL}/Posting`, posting, { headers: this.getHeaders() });
}
deletePost(id: number){
return this._http.delete(`${APIURL}/Posting/${id}`, { headers: this.getHeaders() });
}
getHeaders(){
return new HttpHeaders().set('Authorization', `Bearer ${localStorage.getItem('id_token')}`);
}
}
<file_sep>/src/app/component/userlist/userlist.component.ts
import { Component, OnInit } from '@angular/core';
import { MatTableDataSource } from '@angular/material';
import { User } from 'src/app/models/user';
import { UserService } from 'src/app/services/user.service';
@Component({
selector: 'app-userlist',
templateUrl: './userlist.component.html',
styleUrls: ['./userlist.component.css']
})
export class UserlistComponent implements OnInit {
columnNames = ['First Name', 'Last Name', 'Email', 'Buttons']
dataSource: MatTableDataSource<User>
user: User;
constructor(private _userService: UserService) {
this.getUserRole(); }
ngOnInit() {
this._userService.getUsers().subscribe((users: User[]) => {
this.dataSource = new MatTableDataSource<User>(users);
});
}
getUserRole(){
this._userService.getUser().subscribe((singleUser: User) => {
this.user = singleUser;
console.log(this.user.Role)
})
}
isAdmin(){
if(this.user.Role=='Admin'){
return true;
}
}
}
<file_sep>/src/app/models/organization.ts
export interface Organization {
OrganizationID?: number;
OrganizationName?: string;
OrganizationLink: string;
OrganizationBio: string;
}<file_sep>/src/app/component/postingcreate/postingcreate.component.ts
import { Component, OnInit } from '@angular/core';
import { FormGroup, FormBuilder, FormControl } from '@angular/forms';
import { PostingService } from 'src/app/services/posting.service';
import { Router } from '@angular/router';
import { Organization } from 'src/app/models/organization';
import { MatTableDataSource } from '@angular/material';
import { OrganizationService } from 'src/app/services/organization.service';
export interface Category {
value: string;
viewValue: string;
}
@Component({
selector: 'app-postingcreate',
templateUrl: './postingcreate.component.html',
styleUrls: ['./postingcreate.component.css']
})
export class PostingcreateComponent implements OnInit {
foods: Category[] = [
{value: 'Food', viewValue: 'Food'},
{value: 'Items', viewValue: 'Items'},
{value: 'Services', viewValue: 'Services'}
];
postingForm: FormGroup;
organizations: any;
dataSource: MatTableDataSource<Organization>;
constructor(private _form: FormBuilder, private _postingService: PostingService, private _organizationService: OrganizationService, private _router: Router) {
this.createForm();
}
ngOnInit() {
this._organizationService.getOrganizations().subscribe((organizations: Organization[]) => {
this.dataSource = new MatTableDataSource<Organization>(organizations);
console.log(organizations)
this.organizations = organizations;
})
}
createForm(){
this.postingForm = this._form.group({
title: new FormControl,
details: new FormControl,
address: new FormControl,
city: new FormControl,
state: new FormControl,
nameOfProvider: new FormControl,
category: new FormControl,
dateAvailable: new FormControl
});
}
onSubmit(){
console.log(this.postingForm.value);
this._postingService.createPost(this.postingForm.value).subscribe(data => {
this._router.navigate(['/posting/list']);
});
}
}
<file_sep>/src/app/component/organization/organization-details/organization-details.component.ts
import { Component, OnInit } from '@angular/core';
import { ActivatedRoute } from '@angular/router';
import { OrganizationService } from '../../../services/organization.service';
import { Organization } from '../../../models/organization';
@Component({
selector: 'app-organization-details',
templateUrl: './organization-details.component.html',
styleUrls: ['./organization-details.component.css']
})
export class OrganizationDetailsComponent implements OnInit {
organization: Organization;
constructor(private _activeRoute: ActivatedRoute, private _organizationService: OrganizationService) { }
ngOnInit() {
this._activeRoute.paramMap.subscribe(routeData => {
this._organizationService.getOrganization(routeData.get('id')).subscribe((singleOrganization: Organization) => {
this.organization = singleOrganization;
});
console.log(routeData);
});
}
}
<file_sep>/src/app/component/userdetailsadmin/userdetailsadmin.component.ts
import { Component, OnInit } from '@angular/core';
import { User } from 'src/app/models/user';
import { Posting } from 'src/app/models/posting';
import { UserService } from 'src/app/services/user.service';
import { Router, ActivatedRoute } from '@angular/router';
import { PostingService } from 'src/app/services/posting.service';
@Component({
selector: 'app-userdetailsadmin',
templateUrl: './userdetailsadmin.component.html',
styleUrls: ['./userdetailsadmin.component.css']
})
export class UserdetailsadminComponent implements OnInit {
user: User;
adminUser: User;
posting: Posting
constructor(private _userService: UserService, private _postingService: PostingService, private _router: Router, private _activatedRoute: ActivatedRoute) {
this._activatedRoute.paramMap.subscribe(p=>{
this._postingService.getPostUserDetail(p.get('id')).subscribe((singleUser: User) => {
this.user = singleUser;
console.log(this.user)
this.getUserRole();
})
});
}
ngOnInit() {
}
getUserRole(){
this._userService.getUser().subscribe((userRole: User) => {
this.adminUser = userRole;
console.log(this.adminUser.Role)
})
}
isAdmin(){
if(this.adminUser.Role=='Admin'){
return true;
}
}
}
<file_sep>/src/app/component/organization/organization-create/organization-create.component.ts
import { Component, OnInit } from '@angular/core';
import { FormGroup, FormBuilder, FormControl } from '@angular/forms';
import { OrganizationService } from 'src/app/services/organization.service';
import { Router } from '@angular/router';
import { User } from 'src/app/models/user';
import { UserService } from 'src/app/services/user.service';
@Component({
selector: 'app-organization-create',
templateUrl: './organization-create.component.html',
styleUrls: ['./organization-create.component.css']
})
export class OrganizationcreateComponent implements OnInit {
organizationForm: FormGroup;
user: User;
constructor(private _organizationService: OrganizationService, private _userService: UserService, private _form: FormBuilder, private _router: Router) {
this.createForm();
this.getUserRole();
}
ngOnInit() {
}
createForm() {
this.organizationForm = this._form.group({
OrganizationName: new FormControl,
OrganizationLink: new FormControl,
OrganizationBio: new FormControl
})
}
getUserRole(){
this._userService.getUser().subscribe((singleUser: User) => {
this.user = singleUser;
console.log(this.user.Role)
})
}
isAdmin(){
if(this.user.Role=='Admin'){
return true;
}
}
onSubmit() {
this._organizationService.createOrganization(this.organizationForm.value).subscribe(data => {
this._router.navigate(["/organizations"]);
});
}
}
<file_sep>/src/app/app-routing.module.ts
import{RouterModule, Routes} from '@angular/router'
import { AppComponent } from './app.component'
import { ListofpostingsComponent } from './component/listofpostings/listofpostings.component'
import { LoginComponent } from './component/login/login.component'
import { OrganizationcreateComponent } from './component/organization/organization-create/organization-create.component'
import { OrganizationeditComponent } from './component/organization/organization-edit/organization-edit.component'
import { PasswordchangeComponent } from './component/passwordchange/passwordchange.component'
import { PostingcreateComponent } from './component/postingcreate/postingcreate.component'
import { PostingdetailComponent } from './component/postingdetail/postingdetail.component'
import { PostingeditComponent } from './component/postingedit/postingedit.component'
import { PostingdeleteComponent } from './component/postingdelete/postingdelete.component'
import { RegisterComponent } from './component/register/register.component'
import { UserdetailsComponent } from './component/userdetails/userdetails.component'
import { UserEditComponent } from './component/useredit/useredit.component'
import { UserDeleteComponent } from './component/userdelete/userdelete.component'
import { ContactpageComponent } from './component/contactpage/contactpage.component'
import { NgModule } from '@angular/core';
import { OrganizationindexComponent } from './component/organization/organization-index/organization-index.component';
import { OrganizationdeleteComponent } from './component/organization/organization-delete/organization-delete.component';
import { OrganizationDetailsComponent } from './component/organization/organization-details/organization-details.component';
import { UserlistComponent } from './component/userlist/userlist.component';
import { PostinguserdetailComponent } from './component/postinguserdetail/postinguserdetail.component';
import { UserdeleteadminComponent } from './component/userdeleteadmin/userdeleteadmin.component';
import { HomepageComponent } from './component/homepage/homepage.component'
import { UserdetailsadminComponent } from './component/userdetailsadmin/userdetailsadmin.component';
const routes: Routes = [
{ path: 'register', component: RegisterComponent },
{ path: 'login', component: LoginComponent },
{ path: '', component: HomepageComponent },
{ path: 'posting', children: [
{ path: 'list', component: ListofpostingsComponent },
{ path: 'create', component: PostingcreateComponent },
{ path: 'detail/:id', component: PostingdetailComponent },
{ path: 'edit/:id', component: PostingeditComponent },
{ path: 'delete/:id', component: PostingdeleteComponent },
{ path: 'userdetail/:id', component: PostinguserdetailComponent }
]
},
{
path: 'organizations', children: [
{ path: '', component: OrganizationindexComponent },
{ path: 'create', component: OrganizationcreateComponent },
{ path: 'detail/:id', component: OrganizationDetailsComponent },
{ path: 'edit/:id', component: OrganizationeditComponent },
{ path: 'delete/:id', component: OrganizationdeleteComponent }
]
},
{ path: 'passwordchange', component: PasswordchangeComponent },
{ path: 'postingcreate', component: PostingcreateComponent },
{ path: 'postingdetail', component: PostingdetailComponent },
{ path: 'postingedit', component: PostingeditComponent },
{ path: 'userdetails', component: UserdetailsComponent },
{ path: 'useredit', component: UserEditComponent },
{ path: 'userdelete', component: UserDeleteComponent },
{ path: 'userdeleteadmin/:id', component: UserdeleteadminComponent },
{ path: 'userdetailsadmin/:id', component: UserdetailsadminComponent},
{ path: 'contactpage', component: ContactpageComponent },
{ path: 'userlist', component: UserlistComponent }
// { path: '**', component: AppComponent }
];
@NgModule({
// other imports here
imports: [RouterModule.forRoot(routes)], // {enableTracing: true}
exports: [RouterModule]
})
export class AppRoutingModule { }
<file_sep>/src/app/component/userdeleteadmin/userdeleteadmin.component.ts
import { Component, OnInit } from '@angular/core';
import { User } from 'src/app/models/user';
import { FormGroup, FormBuilder, FormControl } from '@angular/forms';
import { UserService } from 'src/app/services/user.service';
import { Router, ActivatedRoute } from '@angular/router';
import { PostingService } from 'src/app/services/posting.service';
import { Posting } from 'src/app/models/posting';
@Component({
selector: 'app-userdeleteadmin',
templateUrl: './userdeleteadmin.component.html',
styleUrls: ['./userdeleteadmin.component.css']
})
export class UserdeleteadminComponent implements OnInit {
user: User;
adminUser: User;
posting: Posting;
constructor(private _userService:UserService, private _router: Router, private _postingService: PostingService, private _activatedRoute: ActivatedRoute) {
this._activatedRoute.paramMap.subscribe(p=>{
this._postingService.getPostUserDetail(p.get('id')).subscribe((singleUser: User) => {
this.user = singleUser;
console.log(this.user)
// this.createForm();
// console.log(this.user)
this.getUserRole();
console.log(this.user)
})
});
}
// createForm() {
// this.deleteUserForm = this._form.group({
// // FirstName: string;
// // LastName: string;
// // Email: string;
// // PhoneNumber: string;
// UserID: new FormControl(this.user.UserID),
// FirstName: new FormControl(this.user.FirstName),
// LastName: new FormControl(this.user.LastName),
// Email: new FormControl(this.user.Email),
// PhoneNumber: new FormControl(this.user.PhoneNumber)
// });
// }
ngOnInit(){
}
getUserRole(){
this._userService.getUser().subscribe((userRole: User) => {
this.adminUser = userRole;
console.log(this.adminUser.Role)
})
}
isAdmin(){
if(this.adminUser.Role=='Admin'){
return true;
}
}
onDelete(){
console.log(localStorage.getItem('id_token'));
this._userService.deleteUserAdmin(this.user.UserID).subscribe(()=>{
this._router.navigate(['/userlist']);
});
}
}
<file_sep>/src/app/app.module.ts
import { AppRoutingModule } from './app-routing.module';
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { FormsModule, ReactiveFormsModule } from '@angular/forms';
import { HeaderComponent } from './component/header/header.component';
import { MatToolbarModule, MatButtonModule, MatFormFieldModule, MatInputModule, MatTableModule, MatCardModule, MatGridListModule, MatSelectModule } from '@angular/material';
import { RouterModule} from '@angular/router';
import { HttpClientModule } from '@angular/common/http';
import { BrowserAnimationsModule } from '@angular/platform-browser/animations';
import { AppComponent } from './app.component';
import { NavbarComponent } from './component/navbar/navbar.component';
import { FooterComponent } from './component/footer/footer.component';
import { ListofpostingsComponent } from './component/listofpostings/listofpostings.component';
import { PostingdetailComponent } from './component/postingdetail/postingdetail.component';
import { PostingcreateComponent } from './component/postingcreate/postingcreate.component';
import { PostingeditComponent } from './component/postingedit/postingedit.component';
import { OrganizationindexComponent } from './component/organization/organization-index/organization-index.component';
import { OrganizationcreateComponent } from './component/organization/organization-create/organization-create.component';
import { OrganizationeditComponent } from './component/organization/organization-edit/organization-edit.component';
import { OrganizationDetailsComponent } from './component/organization/organization-details/organization-details.component';
import { OrganizationdeleteComponent } from './component/organization/organization-delete/organization-delete.component';
import { LoginComponent } from './component/login/login.component';
import { RegisterComponent } from './component/register/register.component';
import { UserEditComponent } from './component/useredit/useredit.component';
import { UserdetailsComponent } from './component/userdetails/userdetails.component';
import { PasswordchangeComponent } from './component/passwordchange/passwordchange.component';
import { ContactpageComponent } from './component/contactpage/contactpage.component';
import { OrganizationService } from './services/organization.service';
import { PostingService } from './services/posting.service';
import { UserService } from './services/user.service';
import { AuthService } from './services/auth.service';
import { PostingdeleteComponent } from './component/postingdelete/postingdelete.component';
import { UserDeleteComponent } from './component/userdelete/userdelete.component';
import { PersonalizedlistofpostingsComponent } from './component/personalizedlistofpostings/personalizedlistofpostings.component';
import { PostinguserdetailComponent } from './component/postinguserdetail/postinguserdetail.component';
import { UserlistComponent } from './component/userlist/userlist.component';
import { UserdeleteadminComponent } from './component/userdeleteadmin/userdeleteadmin.component';
import { HomepageComponent } from './component/homepage/homepage.component';
import { UserdetailsadminComponent } from './component/userdetailsadmin/userdetailsadmin.component';
@NgModule({
declarations: [
AppComponent,
NavbarComponent,
HeaderComponent,
FooterComponent,
ListofpostingsComponent,
PostingdetailComponent,
PostingcreateComponent,
PostingeditComponent,
OrganizationindexComponent,
OrganizationcreateComponent,
OrganizationeditComponent,
LoginComponent,
RegisterComponent,
UserEditComponent,
UserdetailsComponent,
PasswordchangeComponent,
ContactpageComponent,
HeaderComponent,
PostingdeleteComponent,
UserDeleteComponent,
OrganizationDetailsComponent,
OrganizationdeleteComponent,
PersonalizedlistofpostingsComponent,
PostinguserdetailComponent,
UserlistComponent,
UserdeleteadminComponent,
HomepageComponent,
UserdetailsadminComponent
],
imports: [
BrowserModule,
BrowserAnimationsModule,
HttpClientModule,
MatToolbarModule,
MatButtonModule,
FormsModule,
ReactiveFormsModule,
MatFormFieldModule,
MatInputModule,
MatTableModule,
MatCardModule,
MatGridListModule,
MatFormFieldModule,
MatSelectModule,
AppRoutingModule,
],
providers: [
AuthService,
OrganizationService,
PostingService,
UserService
],
bootstrap: [AppComponent]
})
export class AppModule { }<file_sep>/src/app/component/organization/organization-index/organization-index.component.ts
import { Component, OnInit } from '@angular/core';
import { MatTableDataSource } from '@angular/material';
import { Organization } from '../../../models/organization';
import { OrganizationService } from '../../../services/organization.service';
import { User } from 'src/app/models/user';
import { UserService } from 'src/app/services/user.service';
@Component({
selector: 'app-organization-index',
templateUrl: './organization-index.component.html',
styleUrls: ['./organization-index.component.css']
})
export class OrganizationindexComponent implements OnInit {
columnNames = ['OrganizationName', 'OrganizationBio', 'OrganizationLink', 'buttons' ];
dataSource: MatTableDataSource<Organization>;
user: User;
constructor(private _organizationService: OrganizationService, private _userService: UserService) { }
ngOnInit() {
this._organizationService.getOrganizations().subscribe((organizaions: Organization[]) => {
this.dataSource = new MatTableDataSource<Organization>(organizaions);
console.log(organizaions);
this.getUserRole();
})
}
getUserRole(){
this._userService.getUser().subscribe((singleUser: User) => {
this.user = singleUser;
console.log(this.user.Role)
})
}
isAdmin(){
if(this.user.Role=='Admin'){
return true;
}
}
}
<file_sep>/src/app/component/postingdelete/postingdelete.component.ts
import { Component, OnInit } from '@angular/core';
import { PostingService } from 'src/app/services/posting.service';
import { ActivatedRoute, Router } from '@angular/router';
import { Posting } from 'src/app/models/posting';
@Component({
selector: 'app-postingdelete',
templateUrl: './postingdelete.component.html',
styleUrls: ['./postingdelete.component.css']
})
export class PostingdeleteComponent implements OnInit {
posting: Posting;
constructor(private _postingService: PostingService, private _activatedRoute: ActivatedRoute, private _router: Router) {
this._activatedRoute.paramMap.subscribe(p => {
this._postingService.getPostDetail(p.get('id')).subscribe((singlePost: Posting) => {
this.posting = singlePost;
});
});
}
ngOnInit() {
}
onDelete(){
this._postingService.deletePost(this.posting.PostID).subscribe(() => {
this._router.navigate(['/posting/list'])
});
}
}
<file_sep>/src/app/models/posting.ts
export interface Posting {
PostID?: number;
UserID?: string;
Title: string;
Details: string;
Address: string;
City: string;
State: string;
NameOfProvider: string;
Category: string;
DatePosted?: string;
DateAvailable: Date;
IsCompleted?: boolean;
}<file_sep>/src/app/services/user.service.ts
import { Injectable } from '@angular/core';
import{HttpClient, HttpHeaders} from '@angular/common/http';
import{User} from '../models/user';
import { APIURL } from '../../environments/environment.prod';
// const APIURL = 'https://localhost:44381/';
@Injectable()
export class UserService {
constructor(private _http: HttpClient) { }
getUsers(){
return this._http.get(`${APIURL}api/Account/UserList`, {headers: this.getHeaders()});
}
private getHeaders(){
return new HttpHeaders().set('Authorization', `Bearer ${localStorage.getItem('id_token')}`);
}
getUser(){
return this._http.get(`${APIURL}api/Account/DetailUser`, {headers: this.getHeaders()});
}
updateUser(user: User){
return this._http.put(`${APIURL}api/Account/changeuserinfo`, user, {headers: this.getHeaders()});
}
deleteUser(user: User){
return this._http.put(`${APIURL}api/Account/RemoveUser`, user, {headers: this.getHeaders()});
}
deleteUserAdmin(id: string){
return this._http.put(`${APIURL}api/Account/RemoveUserAdmin?id=${id}`, null, {headers: this.getHeaders()});
}
}
<file_sep>/src/app/component/register/register.component.ts
import { Component, OnInit } from '@angular/core';
import { FormBuilder, FormGroup, FormControl } from '@angular/forms';
import { AuthService } from '../../services/auth.service';
import { Router } from '@angular/router';
// import { Organization } from '../../models/organization'
// import { MatTableDataSource } from '@angular/material';
// import { OrganizationService } from '../../services/organization.service';
@Component({
selector: 'app-register',
templateUrl: './register.component.html',
styleUrls: ['./register.component.css']
})
// export class OrganizationindexComponent implements OnInit {
// columnNames = ['OrganizationName', 'OrganizationBio', 'OrganizationLink', 'buttons' ];
// dataSource: MatTableDataSource<Organization>;
// constructor(private _organizationService: OrganizationService) { }
// ngOnInit() {
// this._organizationService.getOrganizations().subscribe((organizaions: Organization[]) => {
// this.dataSource = new MatTableDataSource<Organization>(organizaions);
// console.log(organizaions)
// })
// }
// }
export class RegisterComponent implements OnInit {
_registerForm: FormGroup;
// organizations: any;
constructor(private _form: FormBuilder, private _authService: AuthService, private _router: Router) {
this.createForm();
}
ngOnInit() {
// this._organizationService.getOrganizations().subscribe((organizations: Organization[]) => {
// this.dataSource = new MatTableDataSource<Organization>(organizations);
// console.log(organizations)
// this.organizations = organizations;
// })
}
createForm(){
this._registerForm = this._form.group({
email: new FormControl,
password: new FormControl,
confirmPassword: new FormControl,
firstName: new FormControl,
lastName: new FormControl,
// OrganizationName: new FormControl,
phoneNumber: new FormControl
});
}
onSubmit(){
console.log(this._registerForm.value);
this._authService
.register(this._registerForm.value)
.subscribe(()=>this._router.navigate(['/login']));
}
}<file_sep>/src/app/component/listofpostings/listofpostings.component.ts
import { Component, OnInit } from '@angular/core';
import { PostingService } from 'src/app/services/posting.service';
import { Posting } from 'src/app/models/posting';
import { MatTableDataSource } from '@angular/material';
import { UserService } from 'src/app/services/user.service';
import { User } from 'src/app/models/user';
@Component({
selector: 'app-listofpostings',
templateUrl: './listofpostings.component.html',
styleUrls: ['./listofpostings.component.css']
})
export class ListofpostingsComponent implements OnInit {
columnNames = ['Title', 'State', 'Available Until', 'buttons']
dataSource: MatTableDataSource<Posting>
currentUser: string;
user: User;
posting: Posting;
constructor(private _postingService: PostingService, private _userService: UserService) { this.getUserID()}
ngOnInit() {
this._postingService.getPostList().subscribe((postings: Posting[]) => {
this.dataSource = new MatTableDataSource<Posting>(postings);
console.log(postings)
});
}
getUserID(){
this._userService.getUser().subscribe((singleUser: User) => {
this.user = singleUser;
console.log(this.user.UserID);
this.currentUser = this.user.UserID;
//console.log(this.posting.UserID)
})
}
// isUser(){
// if (this.posting.UserID == this.user.UserID){
// return true;
// }
// }
}
<file_sep>/src/app/component/userdelete/userdelete.component.ts
import { Component, OnInit } from '@angular/core';
import { UserService } from 'src/app/services/user.service';
import { ActivatedRoute, Router } from '@angular/router';
import { User } from '../../models/user';
import { FormBuilder, FormGroup, FormControl } from '@angular/forms';
@Component({
selector: 'app-userdelete',
templateUrl: './userdelete.component.html',
styleUrls: ['./userdelete.component.css']
})
export class UserDeleteComponent implements OnInit {
user: User;
deleteUserForm: FormGroup;
constructor(private _form: FormBuilder,
private _userService:UserService, private _router: Router) {
this._userService.getUser().subscribe((singleUser: User) => {
this.user = singleUser;
this.createForm();
});
}
createForm() {
this.deleteUserForm = this._form.group({
// FirstName: string;
// LastName: string;
// Email: string;
// PhoneNumber: string;
UserID: new FormControl(this.user.UserID),
FirstName: new FormControl(this.user.FirstName),
LastName: new FormControl(this.user.LastName),
Email: new FormControl(this.user.Email),
PhoneNumber: new FormControl(this.user.PhoneNumber)
});
}
onDelete(){
this._userService.deleteUser(this.user).subscribe(()=>{
this._router.navigate(['/']);
});
}
ngOnInit(){
}
}<file_sep>/src/app/models/userrole.ts
export interface UserRole{
Email: string;
Role: string;
HasRegistered: string;
LoginProvider: null;
} | 2e7e42d7319033529cf34b632ff261b2adb087a4 | [
"TypeScript",
"HTML"
] | 29 | TypeScript | carrielord/FeedTheNeedAngular | 11bea47f7011b67a95e12d8b07bbebcec953c840 | 24d32411ffe2aea304e1c4e0596db7926e7fabc3 | |
refs/heads/master | <file_sep>package es.esy.practikality.post_x;
import android.content.Intent;
import android.graphics.Bitmap;
import android.graphics.Typeface;
import android.net.Uri;
import android.os.Bundle;
import android.provider.MediaStore;
import android.support.v4.content.FileProvider;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.view.WindowManager;
import android.widget.EditText;
import android.widget.ImageView;
import android.widget.RelativeLayout;
import android.widget.TextView;
import android.widget.Toast;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.Calendar;
import java.util.Date;
public class DankIrfan extends AppCompatActivity {
EditText text_top;
EditText text_bottom;
EditText drake_top;
EditText drake_bottom;
ImageView irfan_base_image;
String current_image;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_dank_irfan);
this.getWindow().setFlags(WindowManager.LayoutParams.FLAG_FULLSCREEN, WindowManager.LayoutParams.FLAG_FULLSCREEN);
//customise postx heading
TextView postx_heading = findViewById(R.id.postx_dank_irfan);
postx_heading.setTypeface(Typeface.createFromAsset(getAssets(), "fonts/CaviarDreams.ttf"));
//set_views_to_variables
text_top = findViewById(R.id.dank_irfan_top_text);
text_bottom = findViewById(R.id.dank_irfan_text_bottom);
drake_top = findViewById(R.id.irfan_myboy_top_text);
drake_bottom = findViewById(R.id.irfan_myboy_bottom_text);
current_image = "haveli";
irfan_base_image = findViewById(R.id.irfan_base_image);
//set custom fonts for text views
Typeface impact = Typeface.createFromAsset(getAssets(), "fonts/impact.ttf");
Typeface stilu_Regular = Typeface.createFromAsset(getAssets(), "fonts/stilu-Regular.otf");
text_top.setTypeface(impact);
text_bottom.setTypeface(impact);
drake_top.setTypeface(stilu_Regular);
drake_bottom.setTypeface(stilu_Regular);
//set default irfan meme base image
irfan_base_image.setBackgroundResource(R.drawable.irfan_haveli);
}
//override back to go to LoadTemplates.class
@Override
public void onBackPressed() {
startActivity(new Intent(this, LoadTemplates.class));
finish();
}
//to switch between base images for memes
public void change_image(View view) {
switch (current_image) {
case "haveli":
response_to_base_image_switching(R.drawable.irfan_kid, View.VISIBLE, View.VISIBLE, View.GONE, View.GONE);
current_image = "kid";
break;
case "kid":
response_to_base_image_switching(R.drawable.irfan_myboy, View.GONE, View.GONE, View.VISIBLE, View.VISIBLE);
current_image = "myboy";
break;
case "myboy":
response_to_base_image_switching(R.drawable.irfan_clever, View.VISIBLE, View.VISIBLE, View.GONE, View.GONE);
current_image = "clever";
break;
case "clever":
response_to_base_image_switching(R.drawable.irfan_charlie, View.VISIBLE, View.VISIBLE, View.GONE, View.GONE);
current_image = "charlie";
break;
case "charlie":
default:
response_to_base_image_switching(R.drawable.irfan_haveli, View.VISIBLE, View.GONE, View.GONE, View.GONE);
current_image = "haveli";
break;
}
}
//handle ui responses to base image switching
private void response_to_base_image_switching(int image_name, int text_top_visibility, int text_bottom_visibility, int drake_top_visibility, int drake_bottom_visibility) {
irfan_base_image.setBackgroundResource(image_name);
text_top.setVisibility(text_top_visibility);
text_bottom.setVisibility(text_bottom_visibility);
drake_top.setVisibility(drake_top_visibility);
drake_bottom.setVisibility(drake_bottom_visibility);
}
//share as post
public void share(View view) {
//convert view to Bitmap for sharing
makeToast();
RelativeLayout relativeLayout = findViewById(R.id.irfan_meme_body);
relativeLayout.setDrawingCacheEnabled(true);
relativeLayout.buildDrawingCache(true);
Bitmap bitmap = Bitmap.createBitmap(relativeLayout.getDrawingCache());
relativeLayout.setDrawingCacheEnabled(true);
//save bitmap temporarily in cache
try {
File cachePath = new File(getApplicationContext().getCacheDir(), "images");
boolean if_successful = cachePath.mkdirs();
if (!if_successful) {
System.out.println("Couldn't make the directory");
}
FileOutputStream stream = new FileOutputStream(cachePath + "/image.png"); // overwrites this image every time
bitmap.compress(Bitmap.CompressFormat.PNG, 100, stream);
stream.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
System.out.println("File not found error");
} catch (IOException e) {
e.printStackTrace();
System.out.println("IO Exception error");
}
File imagePath = new File(getApplicationContext().getCacheDir(), "images");
File newFile = new File(imagePath, "image.png");
Uri contentUri = FileProvider.getUriForFile(this, "es.esy.practikality.post_x", newFile);
//create intent for sharing generated bitmap
if (contentUri != null) {
Date dt = Calendar.getInstance().getTime();
MediaStore.Images.Media.insertImage(getContentResolver(), bitmap, dt.toString(), "Post X by Practikality");
Intent shareIntent = new Intent();
shareIntent.setAction(Intent.ACTION_SEND);
shareIntent.addFlags(Intent.FLAG_GRANT_READ_URI_PERMISSION); // temp permission for receiving app to read this file
shareIntent.setDataAndType(contentUri, getContentResolver().getType(contentUri));
shareIntent.putExtra(Intent.EXTRA_STREAM, contentUri);
shareIntent.putExtra(Intent.EXTRA_TEXT, "Shared with #PostXbyPractikality");
shareIntent.putExtra(Intent.EXTRA_SUBJECT, "Shared with #PostXbyPractikality");
startActivity(Intent.createChooser(shareIntent, "Choose an app"));
}
}
//create toasts
private void makeToast() {
Toast.makeText(getApplicationContext(), "Just making those finishing touches", Toast.LENGTH_SHORT).show();
}
}
<file_sep>package es.esy.practikality.post_x;
import android.app.Activity;
import android.content.Context;
import android.content.Intent;
import android.content.SharedPreferences;
import android.graphics.Bitmap;
import android.graphics.Typeface;
import android.net.Uri;
import android.os.Bundle;
import android.provider.MediaStore;
import android.support.v4.content.FileProvider;
import android.support.v7.app.AppCompatActivity;
import android.util.Log;
import android.view.View;
import android.view.WindowManager;
import android.widget.EditText;
import android.widget.ImageView;
import android.widget.LinearLayout;
import android.widget.TextView;
import android.widget.Toast;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.Calendar;
import java.util.Date;
public class TwitterActivity extends AppCompatActivity {
private static final int PICK_FROM_GALLERY = 2;
ImageView profilePicture;
ImageView tweetImage;
boolean profile_picture_clicked;
TextView username;
TextView fullname;
TextView caption;
TextView hashtags;
EditText font_size;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_twitter_template);
this.getWindow().setFlags(WindowManager.LayoutParams.FLAG_FULLSCREEN, WindowManager.LayoutParams.FLAG_FULLSCREEN);
makeToast("Click on profile icon to set an image.");
//customise postx heading
TextView postx_heading = findViewById(R.id.postx_tweet);
postx_heading.setTypeface(Typeface.createFromAsset(getAssets(), "fonts/CaviarDreams.ttf"));
//assign values to variables
profilePicture = findViewById(R.id.profile_picture_of_tweet);
tweetImage = findViewById(R.id.tweet_image);
profile_picture_clicked = false;
username = findViewById(R.id.tweet_username);
fullname = findViewById(R.id.tweet_fullname);
caption = findViewById(R.id.tweet_caption);
hashtags = findViewById(R.id.hashtags_in_tweet);
font_size = findViewById(R.id.tweet_font_size);
//set custom fonts
Typeface stilu_Regular = Typeface.createFromAsset(getAssets(), "fonts/stilu-Regular.otf");
Typeface stilu_Light = Typeface.createFromAsset(getAssets(), "fonts/stilu-Light.otf");
username.setTypeface(stilu_Light);
caption.setTypeface(stilu_Light);
fullname.setTypeface(stilu_Regular);
hashtags.setTypeface(stilu_Light);
//set tweet details stored in Shared Preferences file
SharedPreferences sharedPreferences = getSharedPreferences("postx", Context.MODE_PRIVATE);
String username_value = "@" + sharedPreferences.getString("username", "UserName");
username.setText(username_value);
fullname.setText(sharedPreferences.getString("fullname", "Full Name"));
caption.setText(sharedPreferences.getString("caption_post", "No Caption Found!"));
//place a # symbol before all hashtags (if any)
String stored_hashtags = sharedPreferences.getString("hashtags", "none_found");
if (!stored_hashtags.equals("none_found")) {
String[] hashtags_array = stored_hashtags.split(" ");
StringBuilder stringBuilder = new StringBuilder();
for (String tag : hashtags_array) {
String current_tag = "#" + tag + " ";
stringBuilder.append(current_tag);
}
hashtags.setText(stringBuilder);
} else {
hashtags.setVisibility(View.GONE);
}
}
//update font size if user wants
public void adjustSize(View view) {
String entered_font_size = font_size.getText().toString();
if (entered_font_size.length() > 0) {
caption.setTextSize(Integer.parseInt(entered_font_size));
}
}
//replace the profile picture
public void add_profile_image(View view) {
profile_picture_clicked = true;
Gallery();
}
//add an image to tweet
public void add_tweet_image(View view) {
profile_picture_clicked = false;
Gallery();
}
//choose image for profile picture or to add to the tweet
public void Gallery() {
Intent photoPickerIntent = new Intent(Intent.ACTION_PICK);
photoPickerIntent.setType("image/*");
startActivityForResult(photoPickerIntent, PICK_FROM_GALLERY);
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (resultCode == Activity.RESULT_OK)
switch (requestCode) {
case PICK_FROM_GALLERY:
Uri selectedImage = data.getData();
try {
Bitmap bitmap = MediaStore.Images.Media.getBitmap(getApplicationContext().getContentResolver(), selectedImage);
//check if profile picture was clicked or the add image button
if (profile_picture_clicked) {
profilePicture.setImageBitmap(bitmap);
} else {
tweetImage.setImageBitmap(bitmap);
tweetImage.setVisibility(View.VISIBLE);
}
} catch (IOException e) {
Log.i("TAG", "Some exception " + e);
}
break;
}
}
//share as post
public void share(View view) {
//convert view to Bitmap for sharing
LinearLayout linearLayout = findViewById(R.id.tweet_body);
linearLayout.setDrawingCacheEnabled(true);
linearLayout.buildDrawingCache(true);
Bitmap bitmap = Bitmap.createBitmap(linearLayout.getDrawingCache());
linearLayout.setDrawingCacheEnabled(true);
makeToast("Just making those finishing touches");
//save bitmap temporarily in cache
try {
File cachePath = new File(getApplicationContext().getCacheDir(), "images");
boolean if_successful = cachePath.mkdirs();
if (!if_successful) {
System.out.println("Couldn't make the directory");
}
FileOutputStream stream = new FileOutputStream(cachePath + "/image.png"); // overwrites this image every time
bitmap.compress(Bitmap.CompressFormat.PNG, 100, stream);
stream.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
System.out.println("File not found error");
} catch (IOException e) {
e.printStackTrace();
System.out.println("IO Exception error");
}
File imagePath = new File(getApplicationContext().getCacheDir(), "images");
File newFile = new File(imagePath, "image.png");
Uri contentUri = FileProvider.getUriForFile(this, "es.esy.practikality.post_x", newFile);
//create intent for sharing generated bitmap
if (contentUri != null) {
Date dt = Calendar.getInstance().getTime();
MediaStore.Images.Media.insertImage(getContentResolver(), bitmap, dt.toString(), "Post X by Practikality");
Intent shareIntent = new Intent();
shareIntent.setAction(Intent.ACTION_SEND);
shareIntent.addFlags(Intent.FLAG_GRANT_READ_URI_PERMISSION); // temp permission for receiving app to read this file
shareIntent.setDataAndType(contentUri, getContentResolver().getType(contentUri));
shareIntent.putExtra(Intent.EXTRA_STREAM, contentUri);
shareIntent.putExtra(Intent.EXTRA_TEXT, "Shared with #PostXbyPractikality");
shareIntent.putExtra(Intent.EXTRA_SUBJECT, "Shared with #PostXbyPractikality");
startActivity(Intent.createChooser(shareIntent, "Choose an app"));
finish();
}
}
//create toasts
private void makeToast(String message) {
Toast.makeText(getApplicationContext(), message, Toast.LENGTH_LONG).show();
}
}
<file_sep>### In reference to #`Issue number` [`2-3 word description`]
General Description about what changes you've done
### Screenshot of `screen name`
[Attach the screenshot(s) here]
**Bugs:**
Add in points regarding the bugs you've experienced with your version of the app, if any.
@`mention any of the official Practikality members to review your PR (just one)`
<file_sep># PostX
PostX is the latest development by Practikality Inc. It is a novel post generator and works simply by taking in the general requirements for your post and generating an aesthetically provoking text post which you can share with all your friends and family.

| 944d1138625f416a3b6377f1fd78c01ed6102fd1 | [
"Markdown",
"Java"
] | 4 | Java | Practikality/post-x | 7b0aaabdae52be7747c98baaf473db90be56c7a3 | 8b4bedbd328edeb5509f012ca579ee0e99e091dd | |
refs/heads/master | <repo_name>BearCleverProud/Multilingual-Word-Embedding<file_sep>/create_language_pairs.py
import pickle
import torch
import LoadData
import string
import gensim
class Dict(object):
def __init__(self):
self.word2idx = {}
self.idx2word = {}
self.vocab_size = 0
def extract_words(en2frfile, fr2enfile):
en_dict = {}
fr_dict = {}
threshold = 0.05
with open(en2frfile, "r") as f:
for line in f:
fr, en, prob = line.split()
if float(prob) > threshold:
fr_dict[(fr, en)] = float(prob)
print("Done loading FR to EN dictionary,", len(fr_dict.keys()), "pairs have been loaded")
with open(fr2enfile, "r") as f:
for line in f:
en, fr, prob = line.split()
if float(prob) > threshold:
en_dict[(en, fr)] = float(prob)
print("Done loading EN to FR dictionary,", len(en_dict.keys()), "pairs have been loaded")
return en_dict, fr_dict
if __name__ == "__main__":
# en_dict, fr_dict = extract_words("lex.1.e2f", "lex.1.f2e")
# pickle.dump(en_dict, open("en_dict", "wb"))
# print("Done dumped EN dictionary")
# pickle.dump(fr_dict, open("fr_dict", "wb"))
# print("Done dumped FR dictionary")
en_dict = pickle.load(open("en_dict", "rb"))
print("Done loaded en_dict")
fr_dict = pickle.load(open("fr_dict", "rb"))
print("Done loaded fr_dict")
en_vocab = gensim.models.KeyedVectors.load_word2vec_format("../../polite-dialogue-generation/data/GoogleNews-vectors-negative300.bin", binary=True).vocab.keys()
fr_vocab = torch.load("../code/vocab.fr")
pairs = []
for en, fr in en_dict.keys():
if (len(en) == 1 and en in string.punctuation) or (len(fr) == 1 and fr in string.punctuation) or en == fr:
continue
if fr_dict.get((fr, en)) is not None and en in en_vocab and fr in fr_vocab.word2idx.keys() and en_dict[(en, fr)] * fr_dict[(fr, en)] > 0.1:
print(en, fr, en_dict[(en, fr)], fr_dict[(fr, en)])
pairs.append((en, fr))
print(len(pairs), "of word pairs have been loaded.")
pickle.dump(pairs, open("word_pairs", "wb"))
print("Done dumped word pairs")
<file_sep>/evaluate.py
import torch
if __name__ == "__main__":
word_pair_scores = {}
cos = torch.nn.CosineSimilarity(dim=0, eps=1e-6)
with open("SimLex-999.txt", "r") as f:
for line in f:
if not line.startswith("word1\tword2"):
word1, word2, _, score, _, _, _, _, _, _ = line.split("\t")
word_pair_scores[(word1, word2)] = float(score)
vocab = torch.load("vocab")
Word2Vec = torch.load("Word2Vec_CPU")
correct = 0
total = 0
for word1, word2 in word_pair_scores:
if word1 in vocab.word2idx and word2 in vocab.word2idx:
total += 1
similarity = cos(Word2Vec(torch.LongTensor([vocab.word2idx[word1]])).squeeze(),
Word2Vec(torch.LongTensor([vocab.word2idx[word2]])).squeeze())
if abs(word_pair_scores[(word1, word2)] / 10 - similarity) <= 0.3:
correct += 1
print("Total Accuracy:", correct / total)
<file_sep>/README.md
1. First obtain the word embedding matrix of English and French according to the paper.
2. Run make_en.py and make_fr.py.
3. Get lex.1.e2f and lex.1.f2e from CLSP Grid, and put them in the same directory.
4. Uncomment the comment line of create_language_pairs.py, run the code.
5. Comment the comment line if you would like to speed it up for further use.
6. Run bilingual.py, you can check the argument, and maybe the code position needs to be modified
The rest of them are for training word embeddings, and evaluation of the result of word embedding.
1. Get ant corpus.
2. Run tokenization by Moses.
3. Run remove.py, which removes the name and number, replaced by <name>, <num>.
4. Run window.py, which takes consective 5 words to one line.
5. If you think the corpus is too large, you can always run shrink.py, and specify any size.
6. Then run train.py, there are arguments to be specified as bilingual.py.
7. If you have done training, you can run evaluation.py to evaluate the result.<file_sep>/window.py
w = open("small.fr", "w")
enough = False
count = 0
maximum = 1000000
with open("news.2018.fr.shuffled.tokenized.replace", "r") as f:
for line in f:
line_split = line.split()
line_split.insert(0, "<bos>")
line_split.insert(0, "<bos>")
line_split.append("<eos>")
line_split.append("<eos>")
for i in range(2, len(line_split) - 2):
new_line = line_split[i - 2] + " " + line_split[i - 1] + " " + line_split[i] + " " + line_split[i + 1] + " " + line_split[i + 2] + "\n"
count += 1
if count % 10000 == 0:
print(count)
if count == maximum:
enough = True
break
else:
w.write(new_line)
if enough:
break
w.close()<file_sep>/train.py
import torch.utils.data
import torch.optim
import torch.nn as nn
import torch
import LoadData
import Net
import argparse
import time
import numpy as np
start = time.time()
parser = argparse.ArgumentParser(description='train.py')
parser.add_argument('-epochs', default=100, type=int, help="number of epochs")
parser.add_argument('-batch_size', default=10000, type=int, help="batch size")
parser.add_argument('-embed_dimension', default=200, type=int, help="embedding dimension")
parser.add_argument('-gpus', default=[], nargs='+', type=int,
help="Use CUDA on the listed devices.")
parser.add_argument('-corpus', default="../data/small", type=str, help="corpus to train the model")
opt = parser.parse_args()
device = torch.device("cpu")
if len(opt.gpus) != 0:
torch.cuda.set_device(opt.gpus[0])
device = torch.device("cuda:" + str(opt.gpus[0]))
data_set = LoadData.DataSet(opt.corpus)
num_data = len(data_set)
print("Training instance:", num_data)
dl = torch.utils.data.DataLoader(data_set, batch_size=opt.batch_size, shuffle=True, num_workers=0)
criterion = nn.CrossEntropyLoss().to(device)
epochs = opt.epochs
network = Net.CBOWNet(20000, opt.embed_dimension).to(device)
print(device)
optimizer = torch.optim.Adam(network.parameters())
cos = torch.nn.CosineSimilarity(dim=0, eps=1e-6)
best_loss = 1000000.0
for i in range(epochs):
network.train()
steps = 0
for idx, (data, labels) in enumerate(dl):
data, labels = data.to(device), labels.to(device)
steps += 1
predict = network(data)
loss = criterion(predict, labels)
optimizer.zero_grad()
loss.backward()
optimizer.step()
if steps == 1:
print("Epoch:", i+1, "Steps:", steps, "PPL:", 2 ** loss.item())
if loss.item() < best_loss:
weight = list(network.embed.parameters())[0].data.cpu().numpy()
new_weight = np.zeros_like(weight)
for j in range(len(weight)):
sum = np.sqrt(np.sum(np.square(weight[j])))
new_weight[j] = weight[j] / sum
network.embed.weight.data.copy_(torch.from_numpy(new_weight))
best_loss = loss.item()
torch.save(network.embed, "Word2Vec.fr")
torch.save(network.embed.cpu(), "Word2Vec_CPU.fr")
torch.save(network.embed.weight.cpu().detach().numpy(), "numpy_embed_test.fr")
torch.save(data_set.dic, "vocab.fr")
embed = torch.load("Word2Vec.fr")
dic = torch.load("vocab.fr")
king = torch.LongTensor([dic.word2idx["roi"]]).to(device)
man = torch.LongTensor([dic.word2idx["homme"]]).to(device)
woman = torch.LongTensor([dic.word2idx["femme"]]).to(device)
queen = torch.LongTensor([dic.word2idx["reine"]]).to(device)
king_minus_man_plus_woman = embed(king) - embed(man) + embed(woman)
queen = embed(queen)
output = "Cosine similarity between \"king + man - woman\" and \"queen\": {0:.3f}"
print(output.format(cos(king_minus_man_plus_woman.squeeze(), queen.squeeze()).item()))
paris = torch.LongTensor([dic.word2idx["paris"]]).to(device)
france = torch.LongTensor([dic.word2idx["france"]]).to(device)
uk = torch.LongTensor([dic.word2idx["royaume-uni"]]).to(device)
london = torch.LongTensor([dic.word2idx["londres"]]).to(device)
paris_minus_france_plus_london = embed(paris) - embed(france) + embed(uk)
london = embed(london)
output = "Cosine similarity between \"paris - france + uk\" and \"london\": {0:.3f}"
print(output.format(cos(paris_minus_france_plus_london.squeeze(), london.squeeze()).item()))
network = network.to(device)
<file_sep>/LoadData.py
from torch.utils.data import Dataset
import torch
class Dict(object):
def __init__(self, corpus):
self.idx2word, self.word2idx = build_dic(corpus)
self.vocab_size = len(self.word2idx.keys())
def to_idx(self, word):
return self.word2idx[word]
def to_word(self, idx):
return self.idx2word[idx]
def build_dic(corpus):
word_count = {}
with open(corpus, "r") as f:
for line in f:
line_split = line.split()
for word in line_split:
if word_count.get(word) is None:
word_count[word] = 1
else:
word_count[word] = word_count[word] + 1
count = sorted(word_count.values(), reverse=True)
print(len(count), "words have been loaded")
if len(count) > 20000:
threshold = count[19998]
word_list = []
for word in word_count.keys():
if len(word_list) == 19997:
break
if word_count[word] >= threshold:
word_list.append(word)
words = word_list
else:
words = list(word_count.keys())
words.append("<unk>")
words.append("<BOS>")
words.append("<EOS>")
assert len(words) == 20000
idx2word = {}
word2idx = {}
for i in range(len(words)):
idx2word[i] = words[i]
word2idx[words[i]] = i
return idx2word, word2idx
class DataSet(Dataset):
def __init__(self, corpus):
self.data = open(corpus, 'r').read().strip().split("\n")
for each in self.data:
assert len(each.split()) == 5
self.dic = Dict(corpus)
self.data_size = len(self.data)
def __len__(self):
return len(self.data)
def __getitem__(self, item):
split = self.data[item].split()
replace = []
for each in split:
replace.append(each) if each in self.dic.word2idx.keys() else replace.append("<unk>")
tensor = torch.LongTensor([self.dic.word2idx[replace[0]], self.dic.word2idx[replace[1]],
self.dic.word2idx[replace[3]], self.dic.word2idx[replace[4]]])
return tensor, self.dic.word2idx[replace[2]]
if __name__ == "__main__":
dic = Dict("data/news.2018.en.shuffled.txt")
print(dic.vocab_size)
assert len(dic.word2idx.keys()) == len(dic.idx2word.keys())
<file_sep>/shrink.py
w = open("large.txt", "w")
maximum = 100000000
count = 0
with open("organised", "r") as f:
for line in f:
if line != "\n" and line != "":
count += 1
w.write(line)
if count == maximum:
break
w.close()
# w = open("large", "w")
# with open("large.txt", "r") as f:
# for line in f:
# if line != "\n" and line != "":
# w.write(line)
# w.close()
<file_sep>/remove.py
from nltk.corpus import names
names=[name for name in names.words('male.txt')]+[name for name in names.words('female.txt')]
def digit(strings):
if strings == "." or strings == ",":
return False
for each in strings:
if each not in "0123456789,.":
return False
return True
w = open("news.2018.fr.shuffled.tokenized.replace","w")
with open("news.2018.fr.shuffled.tokenized", "r") as f:
for line in f:
result = ""
scripts = line.split(" ")
for script in scripts:
if script in names:
result += "<person> "
elif digit(script):
result += "<number> "
else:
result += script + " "
w.write(result.lower())
w.close()<file_sep>/Net.py
import torch.nn as nn
import torch
class CBOWNet(nn.Module):
def __init__(self, vocab_size, dimension):
super(CBOWNet, self).__init__()
self.embed = nn.Embedding(vocab_size, dimension)
self.fc1 = nn.Linear(dimension, vocab_size)
# self.argmax = torch.argmax(dim=1)
# self.softmax = nn.LogSoftmax(dim=1)
# self.fc1 = nn.Linear(vocab_size, dimension)
# self.fc2 = nn.Linear(dimension, vocab_size)
def forward(self, x):
x = self.embed(x)
x = x.mean(dim=1)
# x = self.argmax(x)
x = self.fc1(x)
# x = self.softmax(x)
return x
class SkipGramNet(nn.Module):
def __init__(self, vocab_size, dimension):
super(SkipGramNet, self).__init__()
self.fc1 = nn.Linear(vocab_size, dimension)
self.softmax = nn.Softmax(dim=0)
def forward(self, x):
x = self.fc1(x)
x = self.softmax(x)
return x
<file_sep>/bilingual.py
import torch.utils.data
import torch.optim
import torch.nn as nn
import torch
import argparse
import time
import pickle
import numpy as np
# from scipy.spatial.distance import cdist
import gensim
class Dict(object):
def __init__(self):
self.word2idx = {}
self.idx2word = {}
self.vocab_size = 0
class Bilingual(nn.Module):
def __init__(self, en_embed, fr_embed):
super(Bilingual, self).__init__()
self.fc = torch.nn.Linear(en_embed, fr_embed)
self.dropout = torch.nn.Dropout(p=0.4)
def forward(self, x):
x = self.fc(x)
x = self.dropout(x)
return x
def evaluate(en_embed, fr_embed, network, device):
total = len(en_test_words)
correct = 0
net.eval()
en_vecs = en_embed[torch.LongTensor(en_test_idxs)].to(device).squeeze()
en_vecs = network(en_vecs)
# en_vecs = [en_model[w] for w in en_test_words]
# en_vecs = torch.FloatTensor(en_vecs).to(device).squeeze()
# en_vecs = network(en_vecs).squeeze()
fr_vecs = fr_embed[torch.LongTensor(fr_test_idxs)].to(device).squeeze()
cos = torch.nn.CosineSimilarity(dim=0)
highest = -2
idx = -2
print("Start to evaluate")
for j in range(len(en_vecs)):
for k in range(len(fr_vecs)):
result = cos(en_vecs[j], fr_vecs[k])
if result > highest:
highest = result
idx = k
if idx == j:
print(j, str(correct) + "th correct")
correct += 1
highest = -2
idx = -2
# distance = cdist(en_vecs, fr_vecs, metric='cosine')
# distance = np.argmax(distance, axis=0)
# for j in range(len(distance)):
# print(j, distance[j], en_test_words[j], fr_test_words[j], cos(torch.Tensor(en_vecs[j]).squeeze(), torch.Tensor(fr_vecs[j]).squeeze()))
# print(j, distance[j], en_test_words[j], fr_test_words[distance[j]], cos(torch.Tensor(en_vecs[j]).squeeze(), torch.Tensor(fr_vecs[distance[j]]).squeeze()))
# if distance[j] == j:
# correct += 1
#
# predict = network(en_vec)
# similarity = torch.sum(predict.mul(fr_vec), dim=1) / torch.sqrt(torch.sum(predict**2, dim=1))
# for each in similarity:
# if each > 0.5:
# correct += 1
print("Good result percentage:", correct / total)
def orthogonal(matrix):
u, s, vh = np.linalg.svd(matrix)
return u.dot(np.eye(matrix.shape[0])).dot(vh.conjugate())
start = time.time()
parser = argparse.ArgumentParser(description='train.py')
parser.add_argument('-epochs', default=500, type=int, help="number of epochs")
parser.add_argument('-gpus', default=[], nargs='+', type=int,
help="Use CUDA on the listed devices.")
parser.add_argument('-en_dimension', default=300, type=int, help="embedding dimension of English")
parser.add_argument('-fr_dimension', default=300, type=int, help="embedding dimension of French")
parser.add_argument('-partition_ratio', default=0.7, type=float, help="ratio to partition the data")
en_dic = torch.load("en_vocab")
fr_dic = torch.load("vocab.fr")
language_pairs = pickle.load(open("word_pairs", "rb"))
opt = parser.parse_args()
device = torch.device("cpu")
if len(opt.gpus) != 0:
torch.cuda.set_device(opt.gpus[0])
device = torch.device("cuda:" + str(opt.gpus[0]))
en_embed = np.array([torch.load("en_vector_part_1"), torch.load("en_vector_part_2"), torch.load("en_vector_part_3")])
new_en = torch.nn.Embedding(len(en_embed) * en_embed.shape[1], opt.en_dimension)
en_embed = new_en.weight.data.copy_(torch.from_numpy(en_embed).view(-1, opt.en_dimension)).to(device)
# en_model = gensim.models.KeyedVectors.load_word2vec_format('GoogleNews-vectors-negative300.bin', binary=True)
fr_embed = torch.FloatTensor(torch.load("Word2Vec_CPU.fr")).to(device)
net = Bilingual(opt.en_dimension, opt.fr_dimension).to(device)
criterion = nn.CosineEmbeddingLoss().to(device)
optimizer = torch.optim.Adam(net.parameters())
en_idxs = [en_dic.word2idx[en] for en, _ in language_pairs]
fr_idxs = [fr_dic.word2idx[fr] for _, fr in language_pairs]
assert len(en_idxs) == len(fr_idxs)
en_words = [en for en, _ in language_pairs]
data_len = len(fr_idxs)
print("total data number:", data_len)
en_train_words = en_words[0: int(opt.partition_ratio * data_len)]
en_train_idxs = [en_dic.word2idx[each] for each in en_train_words]
en_test_words = en_words[int(0.7 * data_len): int(1 * data_len)]
en_test_idxs = [en_dic.word2idx[each] for each in en_test_words]
fr_train_idxs = fr_idxs[0: int(opt.partition_ratio * data_len)]
fr_test_idxs = fr_idxs[int(0.7 * data_len): int(1 * data_len)]
# en_test_words = [en_dic.idx2word[each] for each in en_test_idxs]
fr_test_words = [fr_dic.idx2word[each] for each in fr_test_idxs]
# en_train_words = [en_dic.idx2word[each] for each in en_train_idxs]
fr_train_words = [fr_dic.idx2word[each] for each in fr_train_idxs]
net.train()
# en_vec = en_embed[torch.LongTensor(en_train_idxs)].to(device).squeeze()
fr_vec = fr_embed[torch.LongTensor(fr_train_idxs)].to(device).squeeze()
en_vec = en_embed[torch.LongTensor(en_train_idxs)].to(device).squeeze()
# en_vec = [en_model[w] for w in en_train_words]
# en_vec = torch.FloatTensor(en_vec).to(device).squeeze()
for i in range(opt.epochs):
pred = net(en_vec)
dot = fr_vec.mul(pred)
loss = - torch.sum(torch.sum(dot, dim=1) / (torch.sqrt(torch.sum(pred ** 2, dim=1))) / (torch.sqrt(torch.sum(fr_vec ** 2, dim=1))))
# loss = criterion(pred, fr_vec, target=torch.ones(pred.shape[0]))
optimizer.zero_grad()
loss.backward()
optimizer.step()
print(i + 1, "epoch, WXZ:", - loss.item())
list(net.parameters())[0].data.copy_(torch.Tensor(orthogonal(list(net.parameters())[0].data.detach().cpu().numpy()))).to(device)
print("Done orthogonal")
evaluate(en_embed, fr_embed, net, device)
torch.save(net, "bilingual.torch")
<file_sep>/make_en.py
import gensim
import torch
import numpy as np
class Dict(object):
def __init__(self):
self.word2idx = {}
self.idx2word = {}
self.vocab_size = 0
en_model = gensim.models.KeyedVectors.load_word2vec_format('../../polite-dialogue-generation/data/GoogleNews-vectors-negative300.bin', binary=True)
dict_ = Dict()
vector = []
i = 0
vocab_size = len(en_model.vocab.keys())
for each in en_model.vocab.keys():
vector.append(en_model[each] / np.sqrt(np.sum(en_model[each] ** 2)))
dict_.word2idx[each] = i
dict_.idx2word[i] = each
i += 1
if i == vocab_size // 3:
torch.save(np.array(vector), "en_vector_part_1", pickle_protocol=4)
vector = []
elif i == 2 * vocab_size // 3:
torch.save(np.array(vector), "en_vector_part_2", pickle_protocol=4)
vector = []
dict_vocab_size = i
torch.save(dict_, "en_vocab")
torch.save(np.array(vector), "en_vector_part_3", pickle_protocol=4)
<file_sep>/requirements.txt
numpy=1.15.4
torch=1.0.1.post2
gensim=3.4.0<file_sep>/source.py
import re
import nltk
nltk.download('brown')
from nltk.corpus import brown
import itertools
import argparse
corpus = []
parser = argparse.ArgumentParser(description='train.py')
parser.add_argument('-batch_size', default=10000, type=int, help="batch size")
parser.add_argument('-embed_dimension', default=100, type=int, help="embedding dimension")
parser.add_argument('-gpu', type=int, help="Use CUDA on the listed devices.")
opt = parser.parse_args()
for cat in ['news']:
for text_id in brown.fileids(cat):
raw_text = list(itertools.chain.from_iterable(brown.sents(text_id)))
text = ' '.join(raw_text)
text = text.lower()
text.replace('\n', ' ')
text = re.sub('[^a-z ]+', '', text)
corpus.append([w for w in text.split() if w != ''])
from collections import Counter
import random, math
def subsample_frequent_words(corpus):
filtered_corpus = []
word_counts = dict(Counter(list(itertools.chain.from_iterable(corpus))))
sum_word_counts = sum(list(word_counts.values()))
word_counts = {word: word_counts[word]/float(sum_word_counts) for word in word_counts}
for text in corpus:
filtered_corpus.append([])
for word in text:
if random.random() < (1+math.sqrt(word_counts[word] * 1e3)) * 1e-3 / float(word_counts[word]):
filtered_corpus[-1].append(word)
return filtered_corpus
corpus = subsample_frequent_words(corpus)
vocabulary = set(itertools.chain.from_iterable(corpus))
word_to_index = {w: idx for (idx, w) in enumerate(vocabulary)}
index_to_word = {idx: w for (idx, w) in enumerate(vocabulary)}
import numpy as np
context_tuple_list = []
w = 4
for text in corpus:
for i, word in enumerate(text):
first_context_word_index = max(0,i-w)
last_context_word_index = min(i+w, len(text))
for j in range(first_context_word_index, last_context_word_index):
if i!=j:
context_tuple_list.append((word, text[j]))
print("There are {} pairs of target and context words".format(len(context_tuple_list)))
import torch
import torch.nn as nn
import torch.autograd as autograd
import torch.optim as optim
import torch.nn.functional as F
device = torch.device("cpu")
if opt.gpu is not None:
device = torch.device("cuda:" + str(opt.gpu))
class Word2Vec(nn.Module):
def __init__(self, embedding_size, vocab_size):
super(Word2Vec, self).__init__()
self.embeddings = nn.Embedding(vocab_size, embedding_size)
self.linear = nn.Linear(embedding_size, vocab_size)
def forward(self, context_word):
emb = self.embeddings(context_word)
hidden = self.linear(emb)
out = F.log_softmax(hidden)
return out
class EarlyStopping():
def __init__(self, patience=5, min_percent_gain=0.1):
self.patience = patience
self.loss_list = []
self.min_percent_gain = min_percent_gain / 100.
def update_loss(self, loss):
self.loss_list.append(loss)
if len(self.loss_list) > self.patience:
del self.loss_list[0]
def stop_training(self):
if len(self.loss_list) == 1:
return False
gain = (max(self.loss_list) - min(self.loss_list)) / max(self.loss_list)
print("Loss gain: {}%".format(round(100*gain,2)))
if gain < self.min_percent_gain:
return True
else:
return False
vocabulary_size = len(vocabulary)
net = Word2Vec(embedding_size=2, vocab_size=vocabulary_size)
loss_function = nn.CrossEntropyLoss()
optimizer = optim.Adam(net.parameters())
early_stopping = EarlyStopping()
context_tensor_list = []
for target, context in context_tuple_list:
target_tensor = autograd.Variable(torch.LongTensor([word_to_index[target]]))
context_tensor = autograd.Variable(torch.LongTensor([word_to_index[context]]))
context_tensor_list.append((target_tensor, context_tensor))
while True:
losses = []
for target_tensor, context_tensor in context_tensor_list:
net.zero_grad()
log_probs = net(context_tensor)
loss = loss_function(log_probs, target_tensor)
loss.backward()
optimizer.step()
losses.append(loss.data)
print("Loss: ", np.mean(losses))
early_stopping.update_loss(np.mean(losses))
if early_stopping.stop_training():
break
import random
def get_batches(context_tuple_list, batch_size=100):
random.shuffle(context_tuple_list)
batches = []
batch_target, batch_context, batch_negative = [], [], []
for i in range(len(context_tuple_list)):
batch_target.append(word_to_index[context_tuple_list[i][0]])
batch_context.append(word_to_index[context_tuple_list[i][1]])
batch_negative.append([word_to_index[w] for w in context_tuple_list[i][2]])
if (i+1) % batch_size == 0 or i == len(context_tuple_list)-1:
tensor_target = autograd.Variable(torch.from_numpy(np.array(batch_target)).long())
tensor_context = autograd.Variable(torch.from_numpy(np.array(batch_context)).long())
tensor_negative = autograd.Variable(torch.from_numpy(np.array(batch_negative)).long())
batches.append((tensor_target, tensor_context, tensor_negative))
batch_target, batch_context, batch_negative = [], [], []
return batches
from numpy.random import multinomial
def sample_negative(sample_size):
sample_probability = {}
word_counts = dict(Counter(list(itertools.chain.from_iterable(corpus))))
normalizing_factor = sum([v**0.75 for v in word_counts.values()])
for word in word_counts:
sample_probability[word] = word_counts[word]**0.75 / normalizing_factor
words = np.array(list(word_counts.keys()))
while True:
word_list = []
sampled_index = np.array(multinomial(sample_size, list(sample_probability.values())))
for index, count in enumerate(sampled_index):
for _ in range(count):
word_list.append(words[index])
yield word_list
import numpy as np
context_tuple_list = []
w = 4
negative_samples = sample_negative(8)
for text in corpus:
for i, word in enumerate(text):
first_context_word_index = max(0,i-w)
last_context_word_index = min(i+w, len(text))
for j in range(first_context_word_index, last_context_word_index):
if i!=j:
context_tuple_list.append((word, text[j], next(negative_samples)))
print("There are {} pairs of target and context words".format(len(context_tuple_list)))
import torch
import torch.nn as nn
import torch.autograd as autograd
import torch.optim as optim
import torch.nn.functional as F
class Word2Vec(nn.Module):
def __init__(self, embedding_size, vocab_size):
super(Word2Vec, self).__init__()
self.embeddings_target = nn.Embedding(vocab_size, embedding_size)
self.embeddings_context = nn.Embedding(vocab_size, embedding_size)
def forward(self, target_word, context_word, negative_example):
emb_target = self.embeddings_target(target_word)
emb_context = self.embeddings_context(context_word)
emb_product = torch.mul(emb_target, emb_context)
emb_product = torch.sum(emb_product, dim=1)
out = torch.sum(F.logsigmoid(emb_product))
emb_negative = self.embeddings_context(negative_example)
emb_product = torch.bmm(emb_negative, emb_target.unsqueeze(2))
emb_product = torch.sum(emb_product, dim=1)
out += torch.sum(F.logsigmoid(-emb_product))
return -out
import time
vocabulary_size = len(vocabulary)
loss_function = nn.CrossEntropyLoss().to(device)
net = Word2Vec(embedding_size=opt.embedding_size, vocab_size=vocabulary_size).to(device)
optimizer = optim.Adam(net.parameters())
early_stopping = EarlyStopping(patience=5, min_percent_gain=1)
while True:
losses = []
context_tuple_batches = get_batches(context_tuple_list, batch_size=opt.batch_size)
for i in range(len(context_tuple_batches)):
net.zero_grad()
target_tensor, context_tensor, negative_tensor = context_tuple_batches[i]
target_tensor, context_tensor, negative_tensor = target_tensor.to(device), context_tensor.to(device), negative_tensor.to(device)
loss = net(target_tensor, context_tensor, negative_tensor)
loss.backward()
optimizer.step()
losses.append(loss.data)
print("Loss: ", np.mean(losses))
early_stopping.update_loss(np.mean(losses))
if early_stopping.stop_training():
break
import numpy as np
def get_closest_word(word, topn=5):
word_distance = []
emb = net.embeddings_target
pdist = nn.PairwiseDistance()
i = word_to_index[word]
lookup_tensor_i = torch.tensor([i], dtype=torch.long)
v_i = emb(lookup_tensor_i)
for j in range(len(vocabulary)):
if j != i:
lookup_tensor_j = torch.tensor([j], dtype=torch.long)
v_j = emb(lookup_tensor_j)
word_distance.append((index_to_word[j], float(pdist(v_i, v_j))))
word_distance.sort(key=lambda x: x[1])
return word_distance[:topn]<file_sep>/make_fr.py
import io
import torch
import numpy as np
class Dict(object):
def __init__(self):
self.word2idx = {}
self.idx2word = {}
self.vocab_size = 0
def load_vectors(fname):
fin = io.open(fname, 'r', encoding='utf-8', newline='\n', errors='ignore')
vocab = Dict()
vector = []
i = 0
for line in fin:
if i == 0:
i += 1
continue
tokens = line.rstrip().split(' ')
fr, vecs = tokens[0], tokens[1:]
vec = np.array([float(component) for component in vecs])
vec /= np.sqrt(np.sum(vec ** 2))
vec = vec.tolist()
vector.append(vec)
vocab.word2idx[fr] = i - 1
vocab.idx2word[i - 1] = fr
vocab.vocab_size = i
i += 1
return vocab, vector
if __name__ == '__main__':
vocab, vector = load_vectors("cc.fr.300.vec")
vector = torch.save(torch.Tensor(vector), "Word2Vec_CPU.fr")
torch.save(vocab, "vocab.fr")
| b96066ac48e96cc4b2e1de3051af607517d29d8c | [
"Markdown",
"Python",
"Text"
] | 14 | Python | BearCleverProud/Multilingual-Word-Embedding | 62d557cca67176a1673d5a888dd9f4dcadb697f1 | 39cb4382895696008b0e19d1acc5d64bf95ab972 | |
refs/heads/master | <repo_name>maheswaranvenkat/rabocustomerstatementprocessing<file_sep>/src/main/java/com/rabo/utils/CommonUtils.java
package com.rabo.utils;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import org.apache.commons.lang3.tuple.ImmutablePair;
import org.apache.commons.lang3.tuple.Pair;
public abstract class CommonUtils {
public static final String CSV_SEPARATOR = ",";
public static <T> Pair<Set<T>,List<T>> resultSet(List<T> all) {
Set<T> set = new HashSet<>();
List<T> duplicateElements=new ArrayList<>();
// Set#add returns false if the set does not change, which
// indicates that a duplicate element has been added.
for (T each: all) if (!set.add(each)) duplicateElements.add(each);
return new ImmutablePair<>(set,duplicateElements);
}
}
<file_sep>/src/main/java/com/rabo/controller/CustomerStatementUploadController.java
package com.rabo.controller;
import com.rabo.business.CSVCustomerStatementProcess;
import com.rabo.business.XMLCustomerStatementProcess;
import com.rabo.exception.CSVProcessingException;
import com.rabo.exception.CustomerStatementProcessException;
import com.rabo.exception.XMLProcessingException;
import com.rabo.model.FileExtensionFilter;
import com.rabo.model.Record;
import com.rabo.service.CustomerStatementErrorReportService;
import java.util.List;
import java.util.Set;
import org.apache.commons.io.FilenameUtils;
import org.apache.commons.lang3.tuple.Pair;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
/**
* Upload Controller is responsible for routing the request to respective Process class and get the output.
*
* @author <NAME>
* @date 18 June 2018
*/
@RestController
public class CustomerStatementUploadController {
@Autowired
CSVCustomerStatementProcess csvCustomerStatementProcess;
@Autowired
XMLCustomerStatementProcess xmlCustomerStatementProcess;
@Autowired
CustomerStatementErrorReportService customerStatementErrorReportService;
@Value("${csvFileName.path}")
private String csvfileNamePath;
@Value("${xmlFileName.path}")
private String xmlfileNamePath;
@Value("${errorFileName.path}")
private String errorDataProcessingFilePath;
@RequestMapping("/")
public String invoke() throws CustomerStatementProcessException, CSVProcessingException, XMLProcessingException {
FileExtensionFilter extensionType = FileExtensionFilter.fromString(FilenameUtils.getExtension(csvfileNamePath));
switch (extensionType) {
case CSV: {
//Thought to have CustomerStatementProvider from where we can get the proper fileName.
Pair<Set<Record>, List<Record>> csvProcessed = csvCustomerStatementProcess.execute(csvfileNamePath);
customerStatementErrorReportService.writeToCSV(csvProcessed.getRight(), errorDataProcessingFilePath);
return "CSV Processed Successfully!!! Please check the Report.csv file is there any errors."
+ " You Can find the file in following location Upload location";
}
case XML: {
Pair<Set<Record>, List<Record>> xmlProcessed = xmlCustomerStatementProcess.execute(xmlfileNamePath);
customerStatementErrorReportService.writeToCSV(xmlProcessed.getRight(), errorDataProcessingFilePath);
return "XML Processed Successfully!!! Please check the Report.csv file is there any errors. "
+ "You Can find the file in following location Upload location";
}
default: {
throw new CustomerStatementProcessException("Customer Doesn't select any related file");
}
}
}
}
<file_sep>/src/main/java/com/rabo/exception/XMLProcessingException.java
package com.rabo.exception;
/**
* Exception class.
*
* @author <NAME>
* @date 18 June 2018
*/
public class XMLProcessingException extends Exception {
private static final long serialVersionUID = 1L;
public XMLProcessingException(String message) {
super(message);
}
}
<file_sep>/src/main/java/com/rabo/model/FileExtensionFilter.java
package com.rabo.model;
import java.util.EnumSet;
import org.apache.commons.lang.StringUtils;
public enum FileExtensionFilter {
CSV("csv"),
XML("xml");
private String code;
FileExtensionFilter(String code) {
this.code = new String(code);
}
public String getCode() {
return code;
}
@Override
public String toString() {
return code;
}
public static FileExtensionFilter fromString(String status) {
return EnumSet.allOf(FileExtensionFilter.class)
.stream()
.filter(value -> StringUtils.equals(value.getCode(), status))
.findFirst()
.orElse(null);
}
}
<file_sep>/src/test/java/com/rabo/AppTest.java
package com.rabo;
import com.rabo.business.CSVCustomerStatementProcess;
import com.rabo.exception.CSVProcessingException;
import com.rabo.exception.CustomerStatementProcessException;
import com.rabo.exception.XMLProcessingException;
import com.rabo.model.FileExtensionFilter;
import org.junit.Test;
import static org.junit.Assert.assertEquals;
import com.rabo.controller.CustomerStatementUploadController;
import org.junit.runner.RunWith;
import org.mockito.Mock;
import org.mockito.Mockito;
import org.mockito.junit.MockitoJUnitRunner;
import org.powermock.core.classloader.annotations.PrepareForTest;
import org.springframework.test.context.TestPropertySource;
import static org.powermock.api.mockito.PowerMockito.mock;
import static org.powermock.api.mockito.PowerMockito.whenNew;
import static org.powermock.api.support.membermodification.MemberMatcher.method;
@RunWith(MockitoJUnitRunner.class)
@TestPropertySource(properties = {
"xmlFileName.path=./upload/records.xml",
})
@PrepareForTest({FileExtensionFilter.class})
public class AppTest {
private CustomerStatementUploadController customerStatementUploadController;
@Mock
private CSVCustomerStatementProcess csvCustomerStatementProcess;
/*@Before
public void setUp() {
fileExtensionFilter = mock(FileExtensionFilter.class);
}*/
@Test
public void testApp() throws CustomerStatementProcessException, CSVProcessingException, XMLProcessingException, Exception {
//Mockito.when(FileExtensionFilter.fromString("./upload/records.xml")).thenReturn(FileExtensionFilter.XML);
//whenNew(FileExtensionFilter.class).withNoArguments().thenReturn(fileExtensionFilter);
//stub(method(FileExtensionFilter.class, "fromString()").toReturn(FileExtensionFilter.XML);
//stub(method(FileExtensionFilter.class, "fromString")).toReturn(FileExtensionFilter.XML);
customerStatementUploadController = Mockito.mock(CustomerStatementUploadController.class, Mockito.CALLS_REAL_METHODS);
String result = customerStatementUploadController.invoke();
assertEquals(result, "CSV Processed Successfully!!! Please check the Report.csv file is there any errors. "
+ "You Can find the file in following location Upload location");
}
}
<file_sep>/src/main/java/com/rabo/business/XMLCustomerStatementProcess.java
package com.rabo.business;
import com.rabo.exception.XMLProcessingException;
import com.rabo.model.Record;
import com.rabo.model.Records;
import com.rabo.utils.CommonUtils;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.List;
import java.util.Set;
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Unmarshaller;
import org.apache.commons.lang3.tuple.Pair;
import org.springframework.stereotype.Component;
/**
* XMLCustomer Statement Process is responsible for processing the data from XML file.
*
* @author <NAME>
* @date 18 June 2018
*/
@Component
public class XMLCustomerStatementProcess {
public Pair<Set<Record>, List<Record>> execute(String xmlFile) throws XMLProcessingException {
Records customerStatementRecords = new Records();
try(final InputStream is = new FileInputStream(new File(xmlFile))) {
JAXBContext jaxbContext = JAXBContext.newInstance(Records.class);
Unmarshaller jaxbUnmarshaller = jaxbContext.createUnmarshaller();
customerStatementRecords = (Records) jaxbUnmarshaller.unmarshal(is);
} catch (JAXBException | IOException e) {
throw new XMLProcessingException("CSV Processing Exception" + e.getMessage());
}
return CommonUtils.resultSet(customerStatementRecords.getRecords());
}
}
| fda0504304ed66ad99e870743937a337819e0a1e | [
"Java"
] | 6 | Java | maheswaranvenkat/rabocustomerstatementprocessing | 945e057c9490c9fe7832af7fc3da4f10cd28cd22 | 2154373d7a3fb057b0117df135908e3d31d32c46 | |
refs/heads/master | <file_sep># Bi-Longitudinal-Unary-Coordinate-Kneading-Algorithm- (B.U.C.K Algorithm)
This algorithm is an experimental exploration into the parallelization of the creation of coordinate loops to be written into
shapefiles. This prototype was written with python using Anaconda 2 on Windows 10. At the time of this readme I am still new to python and this was my first real project using it, so please excuse all the non - pythonic coding.
The inspiration of this algorithm comes from CPU - based 3D rendering techniques called scanline rendering. This algorithm works by reading each row of the raster and creating edglists from the coordinates obtained by picking out the starts and ends of rows of adjacent pixels (segments). These edglists are built sequentially from checking each row of segments against the previous row and inserting into the growing and changing pairs of edgelists through logic taking various cases into account. Each shape is made up of a set of bi-longitudinal edge lists, i.e. an equal number of lists that read sequentially downwards and upwards. An alternate name for this algorithm is the Reverse Scanline algorithm.
The algorithm has four steps and one preliminary step:
- Step 0: Scan the image
- Scan the raster grid row by row and collect arrays of segments. Segments are strings of laterally adjacent pixels of the same value. Group these segments into different tolerances depending on the number of polygon values desired in the final shapefile.
This is the only parallelizable step. i.e: one thread per row, one block (group of threads) per tolerance group.
- Step 1: Buffer segments\Create new shapes
- Iterate through each segment on each row. If this segment is touching another segment on the row above it, it needs to be added to the shape the above segment is a part of. Add this segment to this shapes segment buffer. If this segment is not touching any segment on the row above it, a new shape can be created.
- Step 2: Remove inner loops
- If this segment is touching two or more segments on the row above it that belong to the same shape, an inner loop is created. Extract the loop from the edgelists and put in a separate list within the shape object.
- Step 3: Merge shapes
- If this segment is touching two or more segments on the row above it that belong to different shapes, the shapes need to be merged. Merge each shape needing to be merged one at a time from right to left, merging each shape with the shape to its left until only one shape is left. Due to the loop removal of the previous step, there should be only one segment being touched by the buffered segment per shape.
- Step 4: Add segments
- Once all loops and shape merges caused by this row of segments have been resolved, lines can be transferred from the segment buffer into the edge lists. In the cases of multiple segments touching the same previous segment in the row above, new edglists must be created for that shape.
This algorithm is a prototype and as such does not actually include the aforementioned parallelization and shapefile creation. It can currently only read and construct shape objects composed of edge lists from ASCII Grid files.
<file_sep>import sys
import os
import math
import numpy as np
#import numba as nb
from osgeo import gdal
from PyQt5.QtWidgets import *
from PyQt5.QtGui import *
from PyQt5.uic import *
#The Shape class holds relevant information for loop of coordinates.
#The coordinates are stored as lists, with each list containing an unbroken line moving either upwards
#or downwards. The lists are read in alternating fashion, starting with the first down list, then the first up
#list, then the second down list, etc. There will always be an equal number of up and down lists.
#There is a variable (lastLine) to store the last set of coordinates added and sorted into the lists, and an
#array (segmentBuffer) to store coordinates that are about to be added, and an
#array (innerLoops) of sub loops representing holes in the shape.
class Shape():
def __init__(self, d, u):
self.down = []
self.up = []
self.down.append(d)
self.up.append(u)
#Divided into: innerLoops[ loop[ downLists[ list [ segment[ leftCoord[x,y], rightCoord[x,y] ]+ ]+ ], upLists[ list [ segment[ leftCoord[x,y], rightCoord[x,y] ]+ ]+ ] ]+ ]
self.innerLoops = []
#The last line is divided into interleaving left and right (up and down) coordinates of each segment, without actually putting brackets around each segment
self.lastLine = []
#Divided into: segmentBuffer[ segList[ segment[ leftCoord[x,y], rightCoord[x,y] ]+ ]+ ]
self.segmentBuffer = [0 for x in range(len(self.lastLine)/2)]
#Merge the given shape with this shape. Merge by wrapping the edge pairs of this
#shape around the mergee's edge pairs in a way depending on the index of the connected segments
#in the last line.
#Then merge the segment buffers by appending the tail of the mergee's buffer to this shape's buffer.
def merge(self, s):
#Get index of edge lists to insert merger's edge lists into
insIndex = self.findEdgePair(self.lastLine[-2][0])
#Get index of merger's edge lists to split and rearrange around
splIndex = s.findEdgePair(s.lastLine[0][0])
#Insert merger's down edges from split point into this shape's down edges
self.down[insIndex+1:insIndex+1] = s.down[splIndex+1:]
#Insert merger's up edges from split point into this shape's down edges
self.up[insIndex:insIndex] = s.up[splIndex:]
insIndex += len(s.down[splIndex+1:])
#Insert remaining merger's down edges into this shapes down edges from point after edges prviously added
self.down[insIndex+1:insIndex+1] = s.down[:splIndex+1]
#Insert remaining merger's down edges into this shapes down edges from point after edges prviously added
self.up[insIndex+1:insIndex+1] = s.up[:splIndex]
#Add elements from merging segment buffer to this segment buffer
for elem in s.segmentBuffer[1:]:
self.segmentBuffer.append(elem)
#Append merging shape's inner loops list to this shape's inner loops list
for loop in s.innerLoops:
self.innerLoops.append(loop)
#Put segments in buffer to be added to up/down lists later. This is so we can rearrange edge lists
#due to loop extraction and merging before adding new lines. For each segment in the last
#line, we check if the passed segment is touching it. If it is, we add the segment to its corresponding
#index in a buffer with the same number of elements as segments in the last line.
def bufferSegment(self, segment):
#Check if segment is connected to segment in last line
segDown = segment[0][0] #Leftmost x value of segment
segUp = segment[1][0] #Rightmost x value of segment
leftIdx = 0
#Find last segment left coordinate is connected to and add to correpsonding index in segment buffer
for i, lastSegRight in enumerate(self.lastLine[1::2]):
#Left edge of segment is connected here if left edge position is less than last right edge position
if segDown < lastSegRight[0] and segUp > self.lastLine[i * 2][0]:
#Add to segment buffer, start new array if no other segments connected to this last segment
if self.segmentBuffer[i] == 0:
self.segmentBuffer[i] = [[segment[0], 0]]
else:
self.segmentBuffer[i].append([segment[0], 0])
leftIdx = i * 2
break
else:
return False
#Find last segment right coordinate is connected to and add to correpsonding index in segment buffer
for i, lastSegLeft in enumerate(self.lastLine[-2::-2]):
j = len(self.lastLine)/2 - 1 - i
#Right edge of segment is connected here if right edge position is greater than last left edge position
if segUp > lastSegLeft[0]:
#Add to segment buffer, start new array if no other segments connected to this last segment
if self.segmentBuffer[j] == 0:
self.segmentBuffer[j] = [[0, segment[1]]]
#If right edge of this segment connected to different segment than left edge, we have a loop that needs to be extracted
leftPair = self.findEdgePair(self.lastLine[leftIdx][0])
rightPair = self.findEdgePair(self.lastLine[j * 2][0])
innerEdges = [self.down[(leftPair + 1):(rightPair + 1)], self.up[leftPair:rightPair]]
del self.down[(leftPair + 1):(rightPair + 1)]
del self.up[leftPair:rightPair]
#Also need to reform segmentBuffer
del self.segmentBuffer[leftIdx/2 + 1:j + 1]
self.segmentBuffer[leftIdx/2][-1][1] = segment[1]
#Build loops from extracted inner edges and add to innerLoops
u = 0
d = 1
for edge in innerEdges[0][1:-1]:
if edge[-1] in self.lastLine[::2]:
self.innerLoops.append([innerEdges[0][u:d + 1], innerEdges[1][u:d + 1]])
u = d + 1
d += 1
self.innerLoops.append([innerEdges[0][u:], innerEdges[1][u:]])
#Also need to reform lastLine
self.lastLine[leftIdx + 1] = self.lastLine[j*2+ 1]
del self.lastLine[leftIdx + 2:j*2 + 2]
else:
self.segmentBuffer[j][-1][1] = segment[1]
return True
return False
#Find index of edge pair given x value of left coord
def findEdgePair(self, leftX):
for i, edge in enumerate(self.down):
if leftX == edge[-1][0]:
return i
#Add segments in segment buffer into edge pairs. If more than one segment is connected
#to a segment added in the last line, start a new pair of edge lists
def addLines(self):
if all(x == 0 for x in self.segmentBuffer):
return
#Each index in the buffer corresponds to a possible previous segment that new segments can be attached to
for i, segList in enumerate(self.segmentBuffer):
#Indicies that equal 0 mean there were no segments attached here
if segList == 0:
continue
#Get index of edge pair to recieve segments in list
idx = self.findEdgePair(self.lastLine[i*2][0])
#Append left side of first segment to left edge and right side of last segment to right edge
#If there's only one segment the first and last segments are the same segment
self.down[idx].append(segList[0][0])
self.up[idx].append(segList[-1][1])
#If there's more than one segment, we need to start new edge lists with the unused sides of the
#first and last segments, then add any more segments in between
if len(segList) > 1:
self.up.insert(idx, [ segList[0][1] ])
self.down.insert(idx + 1, [ segList[-1][0] ])
for seg in segList[1:-1]:
self.down.insert(idx + 1, [ seg[0] ])
self.up.insert(idx + 1, [ seg[1] ])
#Finally repopulate last line with new segments and reset segment buffer
self.lastLine = []
for seg in segList:
self.lastLine.append(seg[0])
self.lastLine.append(seg[1])
self.resetBuffer()
#Reset segment buffer by initialzing zeroes equal to half the length of the last line. Each index represents a segment in the last line
def resetBuffer(self):
self.segmentBuffer = [0 for x in range(len(self.lastLine)/2)]
class GPURasterConverter(QDialog):
def __init__(self):
super(GPURasterConverter, self).__init__()
self.initUI()
#Initialize UI
def initUI(self):
loadUi(os.path.join(sys.path[0], 'BUCK_raster_converter_dialog.ui'), self)
#Connect event listeners to functions
self.openPathButton.clicked.connect(self.showOpenDialog)
self.savePathButton.clicked.connect(self.showSaveDialog)
self.tolSlider.valueChanged.connect(self.showTolerance)
self.button_box.accepted.connect(self.execute)
#Render
self.show()
#Prompt user to get path for raster file to open
def showOpenDialog(self):
fname = QFileDialog.getOpenFileName(self, 'Open file', '/home')
self.inputEdit.setText(fname[0])
#Prompt user to set path for output file
def showSaveDialog(self):
fname = QFileDialog.getSaveFileName(self, "Select ouput file", "", "*.shp")
self.outputEdit.setText(fname[0])
#Display tolerance number set by moving slider
def showTolerance(self):
self.valueLabel.setText(str(self.tolSlider.value()))
#Read file and sort coordinates into loops to make polygonal shapefile
def execute(self):
#Get raw array of data from raster file
print("Reading...")
raster = gdal.Open(self.inputEdit.text())
ra2D = raster.ReadAsArray() #2D array
print("Done.")
#Read metadata to help find coordinates of each pixel
file = open(self.inputEdit.text())
cols = float(file.readline().split()[1])
rows = float(file.readline().split()[1])
x = float(file.readline().split()[1]) #Gives x coord of leftmost row
yl = float(file.readline().split()[1]) #Gives y coord of bottom row
cellSize = float(file.readline().split()[1])
noData = float(file.readline().split()[1])
#Convert y coord to top row so can now use top left corner as origin
y = yl + (cellSize * rows)
print("cols: {}rows: {}x: {}y: {}cell size: {}no data value: {}").format(str(cols) + '\n', str(rows) + '\n', str(x) + '\n', str(y) + '\n', str(cellSize) + '\n', str(noData) + '\n')
file.close()
#Put pixel values into groups based on user defined tolerance
groups = int(100/self.tolSlider.value())
print("Groups (based on tolerance): " + str(groups))
max = np.amax(ra2D)
print("Max value: " + str(max))
rasterArray = (ra2D/max) * groups
noData = (noData/max) * groups
print("New no_data value: " + str(int(noData)) + "\n")
# print ("Buffer testing...")
# self.bufferTest()
# print("\nMerge testing...")
# self.mergeTest()
# print("\nAdding lines testing...")
# self.addTest()
#Find coordinates for edge pixels on each line of raster array
print("Scanning for edges...")
scanGroups = [0 for x in range(groups)]
self.scanLines(rasterArray, noData, scanGroups, cellSize, x, y)
print("Finished scanning.\n")
#Build shapes from scanned lines
print("Beginning edge connection...")
shapeGroups = [0 for x in range(groups)]
for i, group in enumerate(scanGroups):
print("Connecting value " + str(i + 1) + " shapes...")
for scanLine in group:
if shapeGroups[i] == 0:
#New to python - couldn't fix contstructor in time
shape = Shape([], [])
shape.down = [ [ scanLine[0][0] ] ]
shape.up = [ [ scanLine[0][1] ] ]
shape.lastLine = [ scanLine[0][0], scanLine[0][1] ]
shape.resetBuffer()
shapeGroups[i] = [shape]
for segment in scanLine[1:]:
shape = Shape([], [])
shape.down = [ [ segment[0] ] ]
shape.up = [ [ segment[1] ] ]
shape.lastLine = [ segment[0], segment[1] ]
shape.resetBuffer()
shapeGroups[i].append(shape)
else:
m = []
for segment in scanLine:
newShape = True
for shape in shapeGroups[i]:
if shape.bufferSegment(segment):
m.append(shape)
if len(m) > 1:
shapeGroups[i].remove(shape)
m[0].merge(shape)
m = [m[0]]
newShape = False
if newShape:
idx = self.findShapeIndex(shapeGroups[i], segment)
shape = Shape([], [])
shape.down = [ [ segment[0] ] ]
shape.up = [ [ segment[1] ] ]
shape.lastLine = [ segment[0], segment[1] ]
shape.resetBuffer()
shapeGroups[i].insert(idx, Shape(segment[0], segment[1]))
for shape in shapeGroups[i]:
shape.addLines()
print("Created " + str(len(shapeGroups[i])) + " shapes")
def findShapeIndex(self, shapeList, segment):
for i, shape in enumerate(shapeList):
if all(x == 0 for x in shape.segmentBuffer):
continue
else:
if segment[0][0] > shape.lastLine[-1][0]:
return i
return len(shapeList) - 1
#Scan rows of raster array and sort edge coordinates into groups depending on tolerance
def scanLines(self, rasterArray, noData, scanGroups, cellSize, originX, originY):
reading = False #Switch to help detmermine what end of the segment we need to look out for
end = len(rasterArray[0]) #Used to help close off segments that are still reading when they get to end of line
for group in range(len(scanGroups)):
print("Scanning value " + str(group + 1) + "...")
size = 0
for i, row in enumerate(rasterArray):
scanLine = []
segment = [0, 0]
for j, col in enumerate(row):
if int(col) == int(noData) and reading == False:
continue
elif math.ceil(col) == group + 1 and reading == False:
reading = True
segment[0] = [originX + (cellSize * j) - (cellSize/2), originY - (cellSize * i)]
elif (math.ceil(col) < group + 1 or i == end - 1) and reading == True:
reading = False
segment[1] = [originX + (cellSize * j) - (cellSize/2), originY - (cellSize * i)]
scanLine.append(segment)
size += 1
segment = [0, 0]
if scanGroups[group] == 0:
scanGroups[group] = scanLine
else:
if scanLine != []:
scanGroups[group].append(scanLine)
print("{} segments, {} lines found").format(size, len(scanGroups[group]))
#=======================TESTING=======================#
#Buffer some segments to a shape
def bufferTest(self):
down = [ [ [0, 0], [0, -1], [0, -2] ], [ [8, -1], [8, -2] ] ]
up = [ [ [3, -1], [3, -2] ], [ [14, 0], [14, -1], [14, -2] ] ]
shape = Shape([], [])
shape.down = down
shape.up = up
shape.lastLine = [ [0, -2], [3, -2], [8, -2], [14, -2] ]
shape.resetBuffer()
passedBuffer = [0, 0]
if not self.checkBuffer(passedBuffer, shape, "Buffer creation test"):
return
segment1 = [ [2, -3], [7, -3] ]
segment2 = [ [9, -3], [10, -3] ]
segment3 = [ [13, -3], [15, -3] ]
shape.bufferSegment(segment1)
passedBuffer = [ [ [ [2, -3], [7, - 3] ] ], 0 ]
if not self.checkBuffer(passedBuffer, shape, "Simple single segment buffer test"):
return
shape.bufferSegment(segment2)
shape.bufferSegment(segment3)
passedBuffer = [ [ [ [2, -3], [7, - 3] ] ], [ [ [9, -3], [10, -3] ], [ [13, -3], [15, -3] ] ] ]
if not self.checkBuffer(passedBuffer, shape, "Simple double segment buffer test"):
return
segment4 = [ [18, -3], [19, -3] ]
segment5 = [ [-3, -3], [-1, -3] ]
shape.bufferSegment(segment4)
shape.bufferSegment(segment5)
if not self.checkBuffer(passedBuffer, shape, "Buffer ignore test"):
return
segment = [ [2, -3], [12, -3] ]
shape.resetBuffer()
shape.bufferSegment(segment)
passedDown = [ [ [0, 0], [0, -1], [0, -2] ] ]
passedUp = [ [ [14, 0], [14, -1], [14, -2] ] ]
passedLoops = [ [ [ [ [8, -1], [8, -2] ] ], [ [ [3, -1], [3, -2] ] ] ] ]
passedBuffer = [ [ [ [2, -3], [12, -3] ] ] ]
if not self.checkLoopRemoval(passedDown, passedUp, passedLoops, shape, "Loop removal test"):
return
if not self.checkBuffer(passedBuffer, shape, "Segment causing loop removal test"):
return
down = [ [ [0, 0], [0, -1], [0, -2], [0, -3] ], [ [7, -1], [7, -2] ], [ [25, -1], [25, -2], [25, -3] ], [ [35, -1], [35, -2], [35, -3] ] ]
up = [ [ [3, -1], [3, -2], [3, -3] ], [ [12, -1], [12, -2] ], [ [30, -1], [30, -2], [30, -3] ], [ [40, 0], [40, -1], [40, -2], [-40, -3] ] ]
shape = Shape([], [])
shape.down = down
shape.up = up
shape.lastLine = [ [0, -3], [3, -3], [25, -3], [30, -3], [35, -3], [40, -3] ]
shape.resetBuffer()
segment = [ [2, -4], [37, -4] ]
shape.bufferSegment(segment)
passedDown = [ [ [0, 0], [0, -1], [0, -2], [0, -3] ] ]
passedUp = [ [ [40, 0], [40, -1], [40, -2], [-40, -3] ] ]
passedLoops = [ [ [ [ [7, -1], [7, -2] ], [ [25, -1], [25, -2], [25, -3] ] ], [ [ [3, -1], [3, -2], [3, -3] ], [ [12, -1], [12, -2] ] ] ], [ [ [ [35, -1], [35, -2], [35, -3] ] ], [ [ [30, -1], [30, -2], [30, -3] ] ] ] ]
passedBuffer = [ [ [ [ 2, -4], [37, -4] ] ] ]
if not self.checkLoopRemoval(passedDown, passedUp, passedLoops, shape, "Multiple loop removal test"):
return
if not self.checkBuffer(passedBuffer, shape, "Segment causing multiple loop removal test"):
return
#Merge two different shapes with a segment between them
def mergeTest(self):
down = [ [0, 0], [0, -1], [0, -2] ]
up = [ [3, 0], [3, -1], [3, -2] ]
shape1 = Shape(down, up)
shape1.lastLine = [ [0, -2], [3, -2] ]
shape1.resetBuffer()
down = [ [6, 0], [6, -1], [6, -2] ]
up = [ [9, 0], [9, -1], [9, -2] ]
shape2 = Shape(down, up)
shape2.lastLine = [ [6, -2], [9, -2] ]
shape2.resetBuffer()
segment = [ [2, -3], [7, -3] ]
shape1.bufferSegment(segment)
shape2.bufferSegment(segment)
shape1.merge(shape2)
passedMergeDown = [ [ [0, 0], [0, -1], [0, -2] ], [ [6, 0], [6, -1], [6, -2] ] ]
passedMergeUp = [ [ [9, 0], [9, -1], [9, -2] ], [ [3, 0], [3, -1], [3, -2] ] ]
passedBuffer = [ [ [ [2, -3], [7, -3] ] ] ]
if not self.checkMerge([], passedMergeDown, passedMergeUp, passedBuffer, shape1, "Simple merge test"):
return
down = [ [ [0, 0], [0, -1], [0, -2] ], [ [10, -1], [10, -2], [10, -3] ], [ [17, -1], [17, -2] ], [ [35, -1], [35, -2], [35, -3] ], [ [45, -1], [45, -2], [45, -3] ], [ [53, -1], [53, -2] ] ]
up = [ [ [3, 0], [3, -1], [3, -2] ], [ [13, -1], [13, -2], [13, -3] ], [ [22, -1], [22, -2] ], [ [40, -1], [40, -2], [40, -3] ], [ [50, -1], [50, -2], [-50, -3] ], [ [57, 0], [57, -1], [57, -2] ] ]
shape1 = Shape([], [])
shape1.down = down
shape1.up = up
shape1.lastLine = [ [10, -3], [13, -3], [35, -3], [40, -3], [45, -3], [50, -3] ]
shape1.resetBuffer()
down = [ [ [60, 0], [60, -1], [60, -2] ], [ [70, 0], [70, -1], [70, -2], [70, -3] ], [ [77, -1], [77, -2] ], [ [95, -1], [95, -2], [95, -3] ], [ [105, -1], [105, -2], [105, -3] ], [ [113, -1], [113, -2] ] ]
up = [ [ [63, 0], [63, -1], [63, -2] ], [ [73, -1], [73, -2], [73, -3] ], [ [82, -1], [82, -2] ], [ [100, -1], [100, -2], [100, -3] ], [ [110, -1], [110, -2], [-110, -3] ], [ [117, 0], [117, -1], [117, -2] ] ]
shape2 = Shape([], [])
shape2.down = down
shape2.up = up
shape2.lastLine = [ [70, -3], [73, -3], [95, -3], [100, -3], [105, -3], [110, -3] ]
shape2.resetBuffer()
segment = [ [37, -4], [97, -4] ]
shape1.bufferSegment(segment)
shape2.bufferSegment(segment)
shape1.merge(shape2)
passedMergeLoops = [ [ [ [ [45, -1], [45, -2], [45, -3] ] ], [ [ [40, -1], [40, -2], [40, -3] ] ] ], [ [ [ [77, -1], [77, -2] ], [ [95, -1], [95, -2], [95, -3] ] ], [ [ [73, -1], [73, -2], [73, -3] ], [ [82, -1], [82, -2] ] ] ] ]
passedMergeDown = [ [ [0, 0], [0, -1], [0, -2] ], [ [10, -1], [10, -2], [10, -3] ], [ [17, -1], [17, -2] ], [ [35, -1], [35, -2], [35, -3] ], [ [105, -1], [105, -2], [105, -3] ], [ [113, -1], [113, -2] ], [ [60, 0], [60, -1], [60, -2] ], [ [70, 0], [70, -1], [70, -2], [70, -3] ], [ [53, -1], [53, -2] ] ]
passedMergeUp = [ [ [3, 0], [3, -1], [3, -2] ], [ [13, -1], [13, -2], [13, -3] ], [ [22, -1], [22, -2] ], [ [100, -1], [100, -2], [100, -3] ], [ [110, -1], [110, -2], [-110, -3] ], [ [117, 0], [117, -1], [117, -2] ], [ [63, 0], [63, -1], [63, -2] ], [ [50, -1], [50, -2], [-50, -3] ], [ [57, 0], [57, -1], [57, -2] ] ]
passedBuffer = [0, [ [ [37, -4], [97, -4] ] ], 0]
if not self.checkMerge(passedMergeLoops, passedMergeDown, passedMergeUp, passedBuffer, shape1, "Complex merge test"):
return
#Add segments to a shape using its segment buffer
def addTest(self):
down = [ [5, 0], [5, -1], [5, -2] ]
up = [ [8, 0], [8, -1], [8, -2] ]
shape = Shape(down, up)
shape.lastLine = [ [5, -2], [8, -2] ]
shape.resetBuffer()
segment = [ [2, -3], [10, -3] ]
shape.bufferSegment(segment)
shape.addLines()
passedDown = [ [ [5, 0], [5, -1], [5, -2], [2, -3] ] ]
passedUp = [ [ [8, 0], [8, -1], [8, -2], [10, -3] ] ]
if not self.checkAdd(passedDown, passedUp, shape, "Single segment add test"):
return
segment1 = [ [3, -4], [6, -4] ]
segment2 = [ [8, -4], [14, -4] ]
shape.bufferSegment(segment1)
shape.bufferSegment(segment2)
shape.addLines()
passedDown = [ [ [5, 0], [5, -1], [5, -2], [2, -3], [3, -4] ], [ [8, -4] ] ]
passedUp = [ [ [6, -4] ], [ [8, 0], [8, -1], [8, -2], [10, -3], [14, -4] ] ]
if not self.checkAdd(passedDown, passedUp, shape, "Double segment add test"):
return
segment1 = [ [1, -5], [4, -5] ]
segment2 = [ [5, -5], [9, -5] ]
segment3 = [ [10, -5], [16, -5] ]
shape.bufferSegment(segment1)
shape.bufferSegment(segment2)
shape.bufferSegment(segment3)
shape.addLines()
passedDown = [ [ [5, 0], [5, -1], [5, -2], [2, -3], [3, -4], [1, -5] ], [ [5, -5] ], [ [10, -5] ] ]
passedUp = [ [ [4, -5] ], [ [9, -5] ], [ [8, 0], [8, -1], [8, -2], [10, -3], [14, -4], [16, -5] ] ]
if not self.checkAdd(passedDown, passedUp, shape, "Triple segment add test"):
return
#Used for buffer insertion scenarios. Check shape buffer against passed buffer then print and return results
def checkBuffer(self, passedBuffer, shape, testString):
if passedBuffer == shape.segmentBuffer:
print(testString + " passed")
return True
else:
print(testString + " failed")
print("Correct buffer: {}\nShape buffer: {}\n").format(passedBuffer, shape.segmentBuffer)
return False
#Used for loop extraction scenarios. Check reordered edge lists and inner loop list against passed variables then print and return results
def checkLoopRemoval(self, passedDown, passedUp, passedLoops, shape, testString):
if passedDown == shape.down and passedUp == shape.up and passedLoops == shape.innerLoops:
print (testString + " passed")
return True
else:
print(testString + " failed")
print("Correct inner loop: {}\nInner loop list: {}\n").format(passedLoops, shape.innerLoops)
print("Correct down edge list: {}\nDown edge list: {}\n").format(passedDown, shape.down)
print("Correct up edge list: {}\nUp edge list: {}\n").format(passedUp, shape.up)
return False
#Used for shape merging scenarios. Check edglists, segment buffer and inner loops against passed variables then print and return results
def checkMerge(self, passedMergeLoops, passedMergeDown, passedMergeUp, passedBuffer, shape, testString):
if shape.down == passedMergeDown and shape.up == passedMergeUp and shape.segmentBuffer == passedBuffer:
print(testString + " passed")
return True
else:
print(testString + " failed")
print("Correct inner loop list: {}\nInner loop list: {}\n").format(passedMergeLoops, shape.innerLoops)
print("Correct down list: {}\nDown list: {}\n").format(passedMergeDown, shape.down)
print("Correct up list: {}\nUp list: {}\n").format(passedMergeUp, shape.up)
print("Correct buffer: {}\nShape buffer: {}\n").format(passedBuffer, shape.segmentBuffer)
return False
#Used for adding segment scenarios. Check edglists against passed edgelists then print and return results
def checkAdd(self, passedDown, passedUp, shape, testString):
if passedDown == shape.down and passedUp == shape.up:
print (testString + " passed")
return True
else:
print(testString + " failed")
print("Correct down edge list: {}\nDown edge list: {}\n").format(passedDown, shape.down)
print("Correct up edge list: {}\nUp edge list: {}\n").format(passedUp, shape.up)
return False
#=======================END TESTING=======================#
if __name__ == '__main__':
app = QApplication(sys.argv)
GPURC = GPURasterConverter()
sys.exit(app.exec_()) | e66e96b946cce335df96f619553e76df20ae5fbd | [
"Markdown",
"Python"
] | 2 | Markdown | MildApocalypse/Bi-Longitudinal-Unary-Coordinate-Kneading-Algorithm- | a7fef2ce1c249fa228cad8ddf44e98855accb7bc | e4d4b2639c136790863fc402ff8d192f9bdcf80d | |
refs/heads/master | <file_sep># spotted-api
An Api for spots locator application
<file_sep>const Mongoose = require('mongoose')
const SpotSchema = Mongoose.Schema({
name: String,
type: String,
description: String,
difficulty: String,
location: {
lat: Number,
lng: Number,
placeId: String,
address: String
},
indoor: Boolean,
media: {
pictures: [String],
videos: [String]
}
}, { collection: 'spots' })
module.exports = Mongoose.model('Spot', SpotSchema)
<file_sep>const spotModel = require('../mongoose/spot.js')
const fakeSpot = require('../fixtures.js')
module.exports = {
getSpots: (req, res) => {
spotModel.find().exec((err, data) => {
if (err) {
res.status(500).send('Something broke : ', err)
}
res.json(data)
})
},
getVisibleSpots: (req, res, corners) => {
const coords = JSON.parse(corners)
spotModel.find({
"localisation.lat": { $gte: coords.swc.lat, $lte: coords.nec.lat },
"localisation.lng": { $gte: coords.swc.lng, $lte: coords.nec.lng },
}).exec((err, data) => {
if (err) {
res.status(500).send(`Something is broken : ${err}`)
}
res.json(data)
})
},
addSpot: (req, res, spot) => {
const newSpot = JSON.parse(spot)
spotModel.create(newSpot, (err, data) => {
if (err) {
res.status(500).send(`Seomething broke : ${err}`)
}
res.status(200).send(`The spot : ${data.name} has been succesfully added !!`)
})
},
updateSpot: (req, res, spot) => {
const newSpot = JSON.parse(spot)
spotModel.updateOne({_id: newSpot._id}, newSpot, (err, data) => {
if (err) {
res.status(500).send(`Something broke : ${err}`)
}
res.status(200).send(`The spot : ${newSpot.name} has been succesfully updated !!`)
})
},
deleteSpot: (req, res, spot) => {
const spotToRemove = JSON.parse(spot)
spotModel.deleteOne(spotToRemove, (err, data) => {
if (err) {
res.status(500).send(`Something broke : ${err}`)
}
res.status(200).send(`The spot : ${spotToRemove._id} has been succesfully deleted !!`)
})
}
}<file_sep>module.exports = {
options: {
host: 'localhost',
SERVER_PORT: 7000,
BDD_URL: 'mongodb://localhost:27017/spotted',
}
}<file_sep>const express = require('express')
const server = express()
const Mongoose = require('mongoose')
const config = require('config')
const spotModel = require('./mongoose/spot.js')
const bdd = config.get('options.BDD_URL')
const routes = require('./routes')
const port = config.get('options.SERVER_PORT')
server.listen(config.get('options.SERVER_PORT'))
console.info('listening on port :', port)
Mongoose.connect(bdd)
routes(server)
<file_sep>const spotsController = require('../controllers')
module.exports = (app) => {
app.get('/api/spots', (req, res) => {
spotsController.getSpots(req, res)
})
app.get('/api/visiblespots/:corners', (req, res) => {
spotsController.getVisibleSpots(req, res, req.params.corners)
})
app.put('/api/addspot/:spot', (req, res) => {
spotsController.addSpot(req, res, req.params.spot)
})
app.put('/api/updatespot/:spot', (req, res) => {
console.log('req.params ===> ', require('util').inspect(req.params, { colors: true, depth: 2 }))
spotsController.updateSpot(req, res, req.params.spot)
})
app.put('/api/deletespot/:spot', (req, res) => {
spotsController.deleteSpot(req, res, req.params.spot)
})
}
| 4fcbcfeedf058195a90b0ba069b04af6652fda4a | [
"Markdown",
"JavaScript"
] | 6 | Markdown | ValJed/spotted-api | bb083b4c68adeb5416688169ca1f76babf0d567a | a03c3ed9799718f503ae4f312854bd937ae3219c | |
refs/heads/master | <repo_name>dkaruli/Profolio<file_sep>/public/js/app.js
var app = angular.module('myport',['ngRoute']);
app.config(function ($routeProvider, $locationProvider) {
$routeProvider
.when('/', {
templateUrl: '../partials/pages/aboutme.html',
controller: 'Home'
})
.when('/Projects', {
templateUrl: '../partials/pages/files.html',
controller: 'FilesController'
})
.when('/Contact', {
templateUrl: '../partials/pages/contacts.html',
controller: 'ContactController'
});
$locationProvider.html5Mode(true);
});
app.directive('navigationMenu', function () {
return {
restrict: 'E',
templateUrl: '../partials/pages/navbar.html',
controller: 'Nav'
};
});
<file_sep>/public/js/Controller/Nav.js
app.controller('Nav', function ($scope,$location,$http,$window){
// Must use a wrapper object, otherwise "activeItem" won't work
$scope.states = {};
$scope.states.activeItem = $location.path();
$scope.items = [{
id: '/',
title: 'Home',
address: "/"
},
{
id: '/Projects',
title: 'Projects',
address: "/Projects"
},
{
id: '/Contact',
title: 'Contact Me',
address: "/Contact"
}];
$scope.source = '/Resume.pdf';
$scope.toggle = false;
/*
$scope.download=function(){
$http.get('resume.pdf', {responseType: "arraybuffer"}).success(function(data){
var arrayBufferView = new Uint8Array( data );
var blob = new Blob( [ arrayBufferView ], { type: 'application/pdf'} );
var urlCreator = window.URL || window.webkitURL;
var imageUrl = urlCreator.createObjectURL( blob );
var img = document.querySelector( "#resume" );
img.src = imageUrl;
SaveToDisk(imageUrl,'resume.pdf');
// window.URL.revokeObjectURL(imageUrl);
// code to download image here
}).error(function(err, status){})
}
function SaveToDisk(fileURL, fileName) {
// for non-IE
if (!window.ActiveXObject) {
var save = document.createElement('a');
save.href = fileURL;
save.target = '_blank';
save.download = fileName || 'unknown';
var event = document.createEvent('Event');
event.initEvent('click', true, true);
save.dispatchEvent(event);
(window.URL || window.webkitURL).revokeObjectURL(save.href);
}
// for IE
else if ( !! window.ActiveXObject && document.execCommand) {
var _window = window.open(fileURL, '_blank');
_window.document.close();
_window.document.execCommand('SaveAs', true, fileName || fileURL)
_window.close();
}
}
*/
});
<file_sep>/public/partials/pages/files.html
<div class="container-fluid">
<div class="row">
<div class="col-xs-6 col-md-3">
<a href="#" class="thumbnail">
<img class="img-responsive" src="penguin.png">
<div class="caption">
<h3>Character Device Driver</h3>
<p>This is Character Device Driver written in C for the Linux Kernel</p>
</div>
</a>
</div>
<div class="col-xs-6 col-md-3">
<a href="#" class="thumbnail">
<img class="img-responsive" src="ImageShare.png">
<div class="caption">
<h3>ImageShare</h3>
<p>iOS Mobile Application written in Swift for photo sharing plateform ImageShare</p>
</div>
</a>
</div>
<div class="col-xs-6 col-md-3">
<a href="#" class="thumbnail">
<img class="img-responsive" src="ImageShare.png">
<div class="caption">
<h3>ImageShare</h3>
<p>Web Application written in AngularJS/HTML5/javascript for photo sharing plateform ImageShare</p>
</div>
</a>
</div>
<div class="col-xs-6 col-md-3">
<a href="#" class="thumbnail">
<img class="img-responsive" src="ImageShare.png">
<div class="caption">
<h3>ImageShare</h3>
<p>Backend written in PHP using Laravel framework for photo sharing plateform ImageShare</p>
</div>
</a>
</div>
</div>
<div class="row">
<div class="col-xs-6 col-md-3">
<a href="#" class="thumbnail">
<img class="img-responsive" src="peg.jpg">
<div class="caption">
<h3>GTA Application Website</h3>
<p>Web Application written in PHP/HTML5/javascript/jQuery for Professors to evaluate new GTA applicants</p>
</div>
</a>
</div>
<div class="col-xs-6 col-md-3">
<a href="#" class="thumbnail">
<img class="img-responsive" src="pacman.png">
<div class="caption">
<h3>Pacman Simulation</h3>
<p>AI simulation of Pacman using Iterative deepening depth-first search algorithm</p>
</div>
</a>
</div>
<div class="col-xs-6 col-md-3">
<a href="#" class="thumbnail">
<img class="img-responsive" src="Hill.png">
<div class="caption">
<h3>Hill cipher</h3>
<p>Implementing the popular Hill cipher encryption using Java</p>
</div>
</a>
</div>
</div>
</div> | ff0b3844d61998fb6e337f1a180df7d9ddf1cf17 | [
"JavaScript",
"HTML"
] | 3 | JavaScript | dkaruli/Profolio | d5b8999c08d78ac37a295dade278507430e4190d | 85d7f2777c67414a5499fc19218f3c27c366404d | |
refs/heads/master | <repo_name>andy120/Tut2<file_sep>/tut2/tut2.cpp
// Comptut2 2015.cpp : Defines the entry point for the console application.
//211543957
//Include libraries
#include <iostream>
#include <cmath>
using namespace std;
//Fraction structure definition
struct Fraction
{
int numerator;
int denominator;
};
//function prototypes
Fraction Create(int, int);
int gcd(int n, int d);
void Display(Fraction);
Fraction Sum(Fraction a, Fraction b);
Fraction Subtract(Fraction a, Fraction b);
Fraction Multiply(Fraction a, Fraction b);
Fraction Divide(Fraction a, Fraction b);
//main function to test program
int main()
{
//declare variables
Fraction a, b, result;
int num, denom;
cout << "Enter fractions in the form [ numerator SPACE denominator] :" << endl << endl;
//prompt user to enter first fraction
cout << "Enter the first fraction :" << endl;
//get value of deneminator and numerator
cin >> num >> denom;
//create fraction
a = Create(num, denom);
//prompt user to eneter next fraction
cout << "Enter the second fraction :" << endl;
//get value of deneminator and numerator
cin >> num >> denom;
//create fraction object
b = Create(num, denom);
//add , subtract ,multiply and divide a and b and display results
result = Sum(a, b);
cout << "The sum of " << a.numerator << "/" << a.denominator << " and " << b.numerator << "/" << b.denominator << " is: ";
Display(result);
result = Subtract(a, b);
cout << "The difference of " << a.numerator << "/" << a.denominator << " and " << b.numerator << "/" << b.denominator << " is: ";
Display(result);
result = Multiply(a, b);
cout << "The product of " << a.numerator << "/" << a.denominator << " and " << b.numerator << "/" << b.denominator << " is: ";
Display(result);
result = Divide(a, b);
cout << "The division of " << a.numerator << "/" << a.denominator << " and " << b.numerator << "/" << b.denominator << " is: ";
Display(result);
//pause display
system("pause");
//show that program executed successfully
return 0;
}
//function to display fractions
void Display(Fraction temp)
{
if (temp.denominator == 1) //check if denominator is 1
cout << temp.numerator << endl;
else if (abs(temp.numerator) > abs(temp.denominator)) //check if fraction is a mixed fraction
cout << temp.numerator / temp.denominator << " " << temp.numerator%temp.denominator << "/" << temp.denominator << endl;
else if (temp.denominator<0)//remove negative sign from denominator
cout << -1 * temp.numerator << "/" << -1 * temp.denominator << endl;
else
cout << temp.numerator << "/" << temp.denominator << endl;
}
//gcd got from Source C#:
int gcd(int n, int d)
{
int remainder;
while (d != 0)
{
remainder = n % d;
n = d;
d = remainder;
}
return n;
}
//function to create fractions
Fraction Create(const int n, const int d)
{
Fraction c;
c.numerator = n;
if (d != 0)
c.denominator = d;
else
{
cout << "Error . Your can not divide by zero" << endl;
exit(0);
}
return c;
}
//function definitions to add , subtract , multiply and divide fractions
Fraction Sum(Fraction a, Fraction b)
{
int n = a.numerator*b.denominator + a.denominator*b.numerator;
int d = a.denominator*b.denominator;
return Create(n / gcd(n, d), d / gcd(n, d));
}
Fraction Subtract(Fraction a, Fraction b)
{
int n = a.numerator*b.denominator - a.denominator*b.numerator;
int d = a.denominator*b.denominator;
return Create(n / gcd(n, d), d / gcd(n, d));
}
Fraction Multiply(Fraction a, Fraction b)
{
int n = a.numerator*b.numerator;
int d = a.denominator*b.denominator;
return Create(n / gcd(n, d), d / gcd(n, d));
}
Fraction Divide(Fraction a, Fraction b)
{
int n = a.numerator*b.denominator;
int d = a.denominator*b.numerator;
return Create(n / gcd(n, d), d / gcd(n, d));
}
| 45f8fc72ef45f04b557d6190185ccaeb6b5d69e8 | [
"C++"
] | 1 | C++ | andy120/Tut2 | 922c7580ec71c564505f3f35f833d0148d2f15fc | 1c0a9e3dff38d9a1038ab0469f0af85dd2d8bbc7 | |
refs/heads/master | <repo_name>chhuovanna/secondhand<file_sep>/app/seller.php
<?php
namespace App;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Support\Facades\DB;
class Seller extends Model
{
//
protected $table ='seller';
protected $primaryKey = 'seller_id';
public static function getSeller($seller_id)
{
$sql = "select * from seller";
return DB::select($sql);
}
public function post()
{
return $this->hasMany('App\Post','seller_id','seller_id');//ADD key
}
public function image(){
return $this->belongsTo('App\Image','image_id','image_id');
}
public static function getSellersWithImage($offset=0){
$sellers = Seller::select(['seller_id', 'name', 'address', 'email','phone','message_account','type','seller.image_id','seller.created_at','seller.updated_at','location','file_name'])
->leftJoin('image','seller.image_id', '=', 'image.image_id')->orderBy('seller.name')->offset($offset)
->limit(04)
->get();
return $sellers;
}
public function user(){
return $this->belongsTo('App\Models\Auth\User','user_id','id');
}
// public static function getactivefeatured($seller_id){
// $sql = <<<EOT
// select date(start_date_time) as start_date, date(end_date_time) as end_date
// from featured_product
// where seller_id = $seller_id and (date(end_date_time) > curdate()
// or date(end_date_time) = '9999-01-01')
// order by updated_at desc
// limit 0, 1;
// EOT;
// return DB::select($sql);
// }
// public static function savefeatured($seller_id, $start_date, $end_date){
// $sql = <<<EOT
// insert into featured_product(product_id, start_date_time, end_date_time)
// values($product_id, '$start_date', '$end_date');
// EOT;
// return DB::insert($sql);
// }
// public static function updatefeatured($product_id, $start_date, $end_date){
// $old_featured = Product::getactivefeatured($product_id);
// $old_featured = $old_featured[0];
// $sql = <<<EOT
// update featured_product set
// start_date_time = '$start_date',
// end_date_time = '$end_date'
// where product_id = $product_id
// and date(start_date_time) = '$old_featured->start_date'
// and date(end_date_time) = '$old_featured->end_date';
// EOT;
// return DB::update($sql);
// }
//
// for seller
public static function getSellersWithThumbnailCategory($offset=0){
$sellers = Seller::select(['seller_id', 'name', 'address', 'email', 'phone'
, 'message_account', 'type', 'seller.image_id', 'seller.created_at'
, 'seller.updated_at', 'location', 'file_name'])
->leftJoin(DB::raw('(select image_id, file_name, location from image) AS temp'), 'seller.image_id', '=', 'temp.image_id')
->with('category')
->offset($offset)
->take(4)
->orderBy('seller.seller_id','desc')
->get();
return $sellers;
}
}
<file_sep>/app/helpers.php
<?php
use App\Helpers\General\Timezone;
use App\Helpers\General\HtmlHelper;
/*
* Global helpers file with misc functions.
*/
if (! function_exists('app_name')) {
/**
* Helper to grab the application name.
*
* @return mixed
*/
function app_name()
{
return config('app.name');
}
}
if (! function_exists('gravatar')) {
/**
* Access the gravatar helper.
*/
function gravatar()
{
return app('gravatar');
}
}
if (! function_exists('timezone')) {
/**
* Access the timezone helper.
*/
function timezone()
{
return resolve(Timezone::class);
}
}
if (! function_exists('include_route_files')) {
/**
* Loops through a folder and requires all PHP files
* Searches sub-directories as well.
*
* @param $folder
*/
function include_route_files($folder)
{
try {
$rdi = new recursiveDirectoryIterator($folder);
$it = new recursiveIteratorIterator($rdi);
while ($it->valid()) {
if (! $it->isDot() && $it->isFile() && $it->isReadable() && $it->current()->getExtension() === 'php') {
require $it->key();
}
$it->next();
}
} catch (Exception $e) {
echo $e->getMessage();
}
}
}
if (! function_exists('home_route')) {
/**
* Return the route to the "home" page depending on authentication/authorization status.
*
* @return string
*/
function home_route()
{
if (auth()->check()) {
if (auth()->user()->can('view backend')) {
return 'admin.dashboard';
} else {
return 'frontend.user.dashboard';
}
}
return 'frontend.index';
}
}
if (! function_exists('style')) {
/**
* @param $url
* @param array $attributes
* @param null $secure
*
* @return mixed
*/
function style($url, $attributes = [], $secure = null)
{
return resolve(HtmlHelper::class)->style($url, $attributes, $secure);
}
}
if (! function_exists('script')) {
/**
* @param $url
* @param array $attributes
* @param null $secure
*
* @return mixed
*/
function script($url, $attributes = [], $secure = null)
{
return resolve(HtmlHelper::class)->script($url, $attributes, $secure);
}
}
if (! function_exists('form_cancel')) {
/**
* @param $cancel_to
* @param $title
* @param string $classes
*
* @return mixed
*/
function form_cancel($cancel_to, $title, $classes = 'btn btn-danger btn-sm')
{
return resolve(HtmlHelper::class)->formCancel($cancel_to, $title, $classes);
}
}
if (! function_exists('form_submit')) {
/**
* @param $title
* @param string $classes
*
* @return mixed
*/
function form_submit($title, $classes = 'btn btn-success btn-sm pull-right')
{
return resolve(HtmlHelper::class)->formSubmit($title, $classes);
}
}
if (! function_exists('camelcase_to_word')) {
/**
* @param $str
*
* @return string
*/
function camelcase_to_word($str)
{
return implode(' ', preg_split('/
(?<=[a-z])
(?=[A-Z])
| (?<=[A-Z])
(?=[A-Z][a-z])
/x', $str));
}
}
<file_sep>/app/Http/Controllers/SellerController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\File; // for deleting file
use Illuminate\Support\Facades\Auth;
use App\Repositories\Frontend\Auth\UserRepository;
use App\Seller;
use App\Image; //need to use it to call new Image();
use Datatables;
use DB;
class SellerController extends Controller
{
/**
* @var UserRepository
*/
protected $userRepository;
/**
* RegisterController constructor.
*
* @param UserRepository $userRepository
*/
public function __construct(UserRepository $userRepository)
{
$this->userRepository = $userRepository;
}
public function index() {
return view('scrud.sellerindex');
}
public function create() {
if (Auth::user()->hasRole('administrator')) {
return view('scrud.sellercreate');
}else{
return redirect()
->back()
->withFlashDanger("You don't have the permission"); // redirect back
}
}
public function store(Request $request) { // add access control if else return you dont have pẻmission
if (Auth::user()->hasRole('administrator')) {
$seller = new Seller();
//$seller->seller_ID = $request->get('seller_id');
$seller->name = $request->get('name');
$seller->address = $request->get('address');
$seller->email = $request->get('email');
$seller->phone = $request->get('phone');
$seller->message_account = $request->get('message_account');
$seller->type = $request->get('type');
//$seller->created_at = $request->get('created_at');
//$seller->updated_at = $request->get('updated_at');
//$seller->image_id = $request->get('image_id');
//validate if the upload file is image
$validatedData = $request->validate([
'image_id' => 'required|image|mimes:jpeg,png,jpg,gif,svg|max:2048',
]);
//get file from input
$file = $request->file('image_id');
$image = new Image();
$image->file_name = rand(1111, 9999) . time() . '.' . $file->getClientOriginalExtension();
$image->location = 'images/seller'; //seller is stored in public/images/seller
try {
$image->save();
//seller the file to it's location on server
$file->move(public_path($image->location), $image->file_name);
//image of seller
$seller->image_id = $image->image_id;
//create new user for selelr
$test = array(
'first_name' => $seller->name,
'last_name' => "",
'email' => $seller->email,
'password' => <PASSWORD>,
'backend' => 1
);
$user = $this->userRepository->create($test);
if ($user){
$user->assignRole('executive');
}
$seller->user_id = $user->id;
$seller->save();
//echo $seller->image_id;
return redirect()->route('seller.index')->withFlashSuccess('seller is added');
} catch (\Exception $e) {
return redirect()
->back()
->withInput($request->all())
->withFlashDanger("Seller can't be added. " . $e->getMessage());
}
}else{
return redirect()
->back()
->withFlashDanger("You don't have the permission"); // redirect back
}
}
public function edit($id) {
if(Auth::user()->hasRole('administrator')){
$seller = Seller::with('image')->find($id);
return view('scrud.selleredit', ['seller' => $seller]);
}else{
$user = Auth::id();
$seller = Seller::where('user_id',$user)->first();
if ($seller->seller_id == $id){
$seller = Seller::with('image')->find($id);
return view('scrud.selleredit', ['seller' => $seller]);
}else{
return redirect()->back()->withFlashDanger("You dont have the permission");
}
}
}
public function update(Request $request, $id) { // add access control
$permit = false;
$changeemail = false;
if (Auth::user()->hasRole('administrator')) {
$permit = true;
}else{
$user = Auth::id();
$seller = Seller::where('user_id',$user)->first();
if ($seller->seller_id == $id){
$permit = true;
}else{
return redirect()
->back()
->withFlashDanger("You dont have the permission " );
}
}
if($permit){
$seller = Seller::find($id);
$seller->seller_id = $request->get('seller_id');
$seller->name = $request->get('name');
$seller->address = $request->get('address');
if ($seller->email != $request->get('email')){
$changeemail = true;
}
$seller->email = $request->get('email');
$seller->phone = $request->get('phone');
$seller->message_account = $request->get('message_account');
$seller->type = $request->get('type');
$seller->created_at = $request->get('created_at');
$seller->updated_at = $request->get('updated_at');
// $seller->image_id = $request->get('image_id');
$validateData = $request->validate([
'image_id' => 'image|mimes:jpeg,png,jpg,gif,svg|max:2048']);
try {
// test if image is updated or not
if ($request->hasFile('image_id')) {
$file = $request->file('image_id');
$image = new Image();
$image->file_name = rand(1111, 9999) . time() . '.' . $file->getClientOriginalExtension();
$image->location = 'images/seller';
$file->move(public_path($image->location), $image->file_name);
$image->save();//save new image
$old_image = $seller->image_id; // Keep the old image for removing if it exists
$seller->image_id = $image->image_id; //change the image to the new one
}
$seller->save();//save the update of category
if($changeemail){
DB::update('update users set email = ? where id = ?', [$seller->email,$seller->user_id]);
}
if (isset($old_image)) {
$old_image = Image::find($old_image);
//remove old image from harddisk
$file = public_path($old_image->location) . '/' . $old_image->file_name;
if (File::exists($file)) {
File::delete($file);
}
$old_image->delete(); //delete the old image if user add a new one
}
return redirect()->route('seller.index')->withFlashSuccess('Seller is updated');
} catch (\Exception $e) {
return redirect()
->back()
->withInput($request->all())
->withFlashDanger("Seller can't be updated. " . $e->getMessage());
}
}
}
public function destroy($id) {
if (Auth::user()->hasRole('administrator')) {
try {
//to get image of the seller to be deleted. in Seller model, there is function called image
$image = Seller::find($id)->image;
//delete seller from database
$res['seller'] = Seller::destroy($id);
if ($image) {
$file = public_path($image->location) . '\\' . $image->file_name;
//test if the image file exists or not
if (File::exists($file)) {
//delete the file from the folder
if (File::delete($file)) {
//delete the image of the seller from database;
$res['image'] = $image->delete();
}
}
}
if ($res['seller'])
return [1];
else
return [0];
} catch (\Exception $e) {
return [0, $e->getMessage()];
}
}else{
return [0, "You don't have the permission"];
}
}
public function getseller(){
if(Auth::user()->hasRole('administrator')) {
//$sellers = seller::select(['seller_id', 'name', 'address', 'email','phone','instant_massage_account','type','seller.created_at','seller.updated_at','seller.image_id','location','file_name']);
$sellers = Seller::select(['seller_id', 'name', 'address', 'email', 'phone', 'message_account', 'type', 'seller.image_id', 'seller.created_at', 'seller.updated_at', 'location', 'file_name'])
->leftJoin(DB::raw('(select image_id, file_name, location from image) AS temp'), 'seller.image_id', '=', 'temp.image_id');
}else{
$user = Auth::id();
$seller = Seller::where('user_id',$user)->first();
$sellers = Seller::select(['seller_id', 'name', 'address', 'email', 'phone'
, 'message_account', 'type', 'seller.image_id', 'seller.created_at'
, 'seller.updated_at', 'location', 'file_name'])
->leftJoin(DB::raw('(select image_id, file_name, location from image) AS temp'), 'seller.image_id', '=', 'temp.image_id')
->where('seller.seller_id',$seller->seller_id);
return Datatables::of($sellers)
->addColumn('action', function ($seller) {
$html = '<a href="'.route('seller.edit', ['seller_id' => $seller->seller_id]).'" class="btn btn-primary btn-sm"><i class="far fa-edit"></i> Edit</a> ';
//$html .= '<a data-id="'.$seller->seller_id.'" class="btn btn-danger btn-sm seller-delete"><i class="far fa-trash-alt"></i></i> Delete</a> ' ;
return $html;
})
->make(true);
}
return Datatables::of($sellers)
->addColumn('action', function ($seller) {
$html = '<a href="'.route('seller.edit', ['seller_id' => $seller->seller_id]).'" class="btn btn-primary btn-sm"><i class="far fa-edit"></i> Edit</a> ';
$html .= '<a data-id="'.$seller->seller_id.'" class="btn btn-danger btn-sm seller-delete"><i class="far fa-trash-alt"></i></i> Delete</a> ' ;
return $html;
})
->make(true);
}
//for shop
public function getsellermore(Request $request){
$sellers = Seller::getSellersWithImage($request->get('offset'));
if(sizeof($sellers) > 0){
$items = array();
foreach ($sellers as $seller){
$html = <<<eot
<div class="col-sm-6 col-md-4 col-lg-3 p-b-35 isotope-item" data-seller_id="$seller->seller_id">
<!-- Block2 -->
<div class="block2" style="height:100%">
<div class="block2-pic hov-img0" >
eot;
if($seller->file_name){
$location = asset($seller->location);
$html .= <<<eot
<img src="$location/$seller->file_name" alt="IMG-SELLER">
eot;
}else{
$location = asset('images/thumbnail');
$html .= <<<eot
<img src="$location/default.png" alt="IMG-SELLER">
eot;
}
$location = asset('cozastore');
$url = route('frontend.product.showbyshop', $seller->seller_id);
$html .= <<<eot
<a href="$url" class="block2-btn flex-c-m stext-103 cl2 size-102 bg0 bor2 hov-btn1 p-lr-15 trans-04 show-product-shop" data-seller_id="$seller->seller_id">
View Store
</a>
</div>
<div class="block2-txt flex-w flex-t p-t-14">
<div class="block2-txt-child1 flex-col-l ">
<span class="stext-105 cl3 ">
<b class="sname">Name: $seller->name</b>
</span>
<span class="stext-105 cl3 address">
<strong>Address: </strong>$seller->address
</span>
<span class="stext-105 cl3 email">
<strong>Email: </strong>$seller->email
</span>
<span class="stext-105 cl3 phone">
<strong>Phone: </strong>$seller->phone
</span>
<span class="stext-105 cl3 message_account">
<strong>Message Account: </strong>$seller->message_account
</span>
<span class="stext-105 cl3 type">
<strong>Type: </strong>$seller->type
</span>
</div>
</div>
</div>
</div>
eot;
$items[] = $html;
}
//return [1,$html];
$totalSize = Seller::count();
return [1,$totalSize,$items];
}
else{
$totalSize = Seller::count();
return [0,$totalSize];
}
}
}
<file_sep>/routes/web.php
<?php
use App\Http\Controllers\LanguageController;
/*
* Global Routes
* Routes that are used between both frontend and backend.
*/
// Switch between the included languages
Route::get('lang/{lang}', [LanguageController::class, 'swap']);
/*
* Frontend Routes
* Namespaces indicate folder structure
*/
Route::group(['namespace' => 'Frontend', 'as' => 'frontend.'], function () {
include_route_files(__DIR__.'/frontend/');
});
/*
* Backend Routes
* Namespaces indicate folder structure
*/
Route::group(['namespace' => 'Backend', 'prefix' => 'admin', 'as' => 'admin.', 'middleware' => 'admin'], function () {
/*
* These routes need view-backend permission
* (good if you want to allow more than one group in the backend,
* then limit the backend features by different roles or permissions)
*
* Note: Administrator has all permissions so you do not have to specify the administrator role everywhere.
* These routes can not be hit if the password is expired
*/
include_route_files(__DIR__.'/backend/');
});
//Route::get ('edit','EditcategoryController@editcategory');
//Route::get('delete','DeletecategoryController@deletecategory');
//Route::get('category','CategoryController@category');
//Route::get('admin/category/rate','CategoryController@getform')->name('category.rate');
//Route::post('admin/category/saveseller','CategoryController@saveseller');
//Route::get('admin/category/showrate','CategoryController@showrate')->name('category.showrate');
//Route::get('admin/category/getseller','CategoryController@getseller')->name('category.getcategory');
Route::get('admin/category/getseller','SellerController@getseller')->name('seller.getseller');
Route::get('admin/category/getcategory', 'CategoryController@getcategory')->name('category.getcategory');
Route::get('admin/category/getproduct', 'ProductController@getproduct')->name('product.getproduct');
//Route::get('signup','Sign_upController@signup');
//Route::post('signup','Sign_upController@postSignup');
Route::get('admin/category/getcategorymore', 'CategoryController@getcategorymore');
Route::get('admin/category/getsellermore', 'SellerController@getsellermore');
Route::get('admin/product/createwithpost/{post}', 'ProductController@createwitholdpost')->name('product.create.with.oldpost');;
Route::get('admin/product/getphotos', 'ProductController@getphotos');
Route::get('admin/product/getactivefeatured','ProductController@getactivefeatured');
Route::get('admin/product/savefeatured','ProductController@savefeatured');
Route::get('admin/product/getproductmore','ProductController@getproductmore');
Route::get('admin/seller/getsellermore','SellerController@getsellermore');
Route::get('admin/product/getproductdetail','ProductController@getproductdetail');
Route::get('admin/message/listmessage', 'MessageController@index')->name('message.listmessage');
Route::get('admin/message/getmessage', 'MessageController@getmessage')->name('message.getmessage');
Route::get('admin/message/markread', 'MessageController@markread')->name('message.markread');
Route::get('admin/message/getUnread', 'MessageController@getUnread');
Route::get('admin/product/likeUnlike', 'ProductController@likeUnlike');
Route::get('admin/product/getproductmorefeature','ProductController@getproductmorefeature');
Route::resource('admin/category','CategoryController');
Route::resource('admin/seller','SellerController');
Route::resource('admin/product','ProductController');
<file_sep>/database/migrations/2019_05_01_073834_create_seller_table.php
<?php
use Illuminate\Support\Facades\Schema;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Database\Migrations\Migration;
class CreateSellerTable extends Migration
{
public function up()
{
Schema::create('seller', function (Blueprint $table) {
$table->increments('seller_id');
$table->string('name')->unique();
$table->string('address')->nullable();
$table->string('email')->nullable();
$table->string('phone')->nullable();
$table->string('message_account')->nullable();
$table->string('type')->nullable();
$table->dateTime('created_at')->default(DB::raw('CURRENT_TIMESTAMP'));
$table->dateTime('updated_at')->default(DB::raw('CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP'));
$table->integer('user_id')->unsigned()->nullable();
$table->integer('image_id')->unsigned()->nullable();
$table->foreign('image_id')->references('image_id')->on('image');
$table->foreign('user_id')->references('id')->on('users');
$table->engine = 'InnoDB';
});
}
public function down()
{
Schema::drop('seller');
}
}
<file_sep>/app/image.php
<?php
namespace App;
use Illuminate\Database\Eloquent\Model;
class Image extends Model
{
protected $table ='image';
protected $primaryKey = 'image_id';
public function product()
{
return $this->belongsTo('App\Product','product_id',' product_id'); //not seller
}
public function category()
{
return $this->hasOne('App\Category', 'image_id', 'image_id');
}
public function seller()
{
return $this->hasOne('App\Seller','image_id','image_id');
}
}
<file_sep>/resources/js/backend/app.js
import '@coreui/coreui'
import 'decimal.js'
import "datatables.net"
import "datatables.net-dt"
import "select2"
import "lightgallery"
import "lg-thumbnail"
import "lg-fullscreen"
<file_sep>/database/migrations/2019_05_03_081926_create_product_table.php
<?php
use Illuminate\Support\Facades\Schema;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Database\Migrations\Migration;
class CreateProductTable extends Migration
{
public function up()
{
Schema::create('product', function (Blueprint $table) {//create product not seller
$table->increments('product_id');
$table->string('name');
$table->decimal('price');
$table->text('description')->nullable();
$table->integer('like_number')->nullable();
$table->text('status')->nullable();
$table->text('pickup_address')->nullable();
$table->text('pickup_time')->nullable();
$table->dateTime('created_at')->default(DB::raw('CURRENT_TIMESTAMP'));
$table->dateTime('updated_at')->default(DB::raw('CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP'));
$table->integer('post_id')->unsigned()->nullable();
$table->foreign('post_id')->references('post_id')->on('post');
$table->integer('image_id')->unsigned()->nullable();
$table->foreign('image_id')->references('image_id')->on('image');
$table->engine = 'InnoDB';
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::drop('product');
}
}
<file_sep>/routes/breadcrumbs/backend/message.php
<?php
Breadcrumbs::for('message.listmessage', function ($trail) {
$trail->push( 'List message', route('message.listmessage'));
});
<file_sep>/database/seeds/AboutTableSeeder.php
<?php
use Illuminate\Database\Seeder;
/**
* Class AboutTableSeeder.
*/
class AboutTableSeeder extends Seeder
{
use TruncateTable;
/**
* Run the database seeds.
*
* @return void
*/
public function run()
{
App\About::truncate();
DB::table('about')->insert([
'phone' => '012345678'
,'email' => '<EMAIL>'
,'website' => 'www.shop.com'
,'address' => '#123, st. 45, Phnom Penh, Cambodia'
]);
}
}
<file_sep>/database/seeds/SellerTableSeeder.php
<?php
use Illuminate\Database\Seeder;
/**
* Class AboutTableSeeder.
*/
class SellerTableSeeder extends Seeder
{
use TruncateTable;
/**
* Run the database seeds.
*
* @return void
*/
public function run()
{
//App\Seller::truncate();
DB::table('seller')->insert([
"name" => "<NAME>"
,'phone' => '012345678'
,'email' => '<EMAIL>'
,'message_account' => '<EMAIL>'
,'address' => '#123, st. 45, Phnom Penh, Cambodia'
,'type' => 'Individual'
,'user_id' => '1'
]);
}
}
<file_sep>/routes/breadcrumbs/backend/seller.php
<?php
Breadcrumbs::for('seller.index', function ($trail) {
$trail->push( 'List seller', route('seller.index'));
});
Breadcrumbs::for('seller.create', function ($trail) {
$trail->parent('seller.index');
$trail->push( 'Create seller', route('seller.create'));
});
Breadcrumbs::for('seller.create.with.oldpost', function ($trail) {
$trail->parent('seller.index');
$trail->push( 'Create seller', route('seller.create'));
});
Breadcrumbs::for('seller.edit', function ($trail,$id) {
$trail->parent('seller.index');
$trail->push( 'Create seller', route('seller.edit',$id));
});
<file_sep>/app/category.php
<?php
namespace App;
use Illuminate\Database\Eloquent\Model;
class Category extends Model
{
//
protected $table ='category';
protected $primaryKey = 'category_id';
public function product()
{
return $this->belongsToMany('App\Product','product_category','category_id','product_id');//not seller ; add intermediate table and key name
}
public function thumbnail()
{
return $this->belongsTo('App\Image','image_id','image_id');
}
public static function getCategorysWithImage($offset=0){
$categorys = Category::select(['category_id', 'name', 'description'
,'category.image_id','category.created_at','category.updated_at'
,'location','file_name'])
->leftJoin('image','category.image_id', '=', 'image.image_id')->orderBy('category.category_id','desc')->offset($offset)
->limit(20)->get();
return $categorys;
}
public static function getSelectOptions(){
$rows = Category::all();
$result = [];
foreach ($rows as $row) {
$id = $row->category_id;
$name = $row->name;
$result[$id] = "$id:$name";
}
return $result;
}
}
<file_sep>/app/Http/Controllers/CategoryController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\File;//for deleting file
use Illuminate\Support\Facades\Auth;
use App\Category;
use App\Image; //need to use it to call new Image();
use Datatables;
use DB;
class CategoryController extends Controller
{
public function index() {
return view('scrud.categoryindex');
}
public function create()
{
if (Auth::user()->hasRole('administrator')) {
return view('scrud.categorycreate');
}else{
return redirect()
->back()
->withFlashDanger("You don't have the permission");
}
}
public function store(Request $request)
{ //add access control
if (Auth::user()->hasRole('administrator')) {
$category = new Category();
//$category->category_id = $request->get('category_id');
$category->name = $request->get('name');
$category->description = $request->get('description');
//$category->image_id = $request->get('image_id');
//validate if the upload file is image
$validateData = $request->validate([
'image_id' => 'required|image|mimes:jpeg,png,jpg,gif,svg|max:2048']);
//get file from input
$file = $request->file('image_id');
$image = new Image();
$image->file_name = rand(1111, 9999) . time() . '.' . $file->getClientOriginalExtension();
$image->location = 'images/category'; //category is stored in public/images/category
try {
$image->save();
//category the file to it's location on server
$file->move(public_path($image->location), $image->file_name);
//image of category
$category->image_id = $image->image_id;
$category->save();
//echo $category->image_id;
return redirect()->route('category.index')->withFlashSuccess('Category is added');
} catch (\Exception $e) {
return redirect()
->back()
->withInput($request->all())
->withFlashDanger("Category can't be added. " . $e->getMessage());
}
}else{
return "You don't have the permission";
}
}
// public function show($id) {
// echo 'show';
// }
public function edit($id) {
if (Auth::user()->hasRole('administrator')) {
//we dont have image function in model category, we only have thumbnail()
$category = Category::with('thumbnail')->find($id);
return view('scrud.categoryedit', ['category' => $category]);
}else{
return redirect()
->back()
->withFlashDanger("You don't have the permission");
}
}
public function update(Request $request, $id)
{ // add access control
if (Auth::user()->hasRole('administrator')) {
$category = Category::find($id);
//$category->category_id = $request->get('category_id');
$category->name = $request->get('name');
$category->description = $request->get('description');
//$category->image_id = $request->get('image_id');
//$category->created_at = $request->get('created_at');
//$category->updated_at = $request->get('updated_at');
$validateData = $request->validate([
'image_id' => 'image|mimes:jpeg,png,jpg,gif,svg|max:2048']);
try {
// test if image is updated or not
if ($request->hasFile('image_id')) {
$file = $request->file('image_id');
$image = new Image();
$image->file_name = rand(1111, 9999) . time() . '.' . $file->getClientOriginalExtension();
$image->location = 'images/category';
$file->move(public_path($image->location), $image->file_name);
$image->save();//save new image
$old_image = $category->image_id; // Keep the old image for removing if it exists
$category->image_id = $image->image_id; //change the image to the new one
}
$category->save(); //save the update of seller
if (isset($old_image)) {
$old_image = Image::find($old_image);
//remove old image from harddisk
$file = public_path($old_image->location) . '/' . $old_image->file_name;
if (File::exists($file)) {
File::delete($file);
}
$old_image->delete(); //delete the old image if user add a new one
}
return redirect()->route('category.index')->withFlashSuccess('Category is updated');
} catch (\Exception $e) {
return redirect()
->back()
->withInput($request->all())
->withFlashDanger("Category can't be updated. " . $e->getMessage());
}
}else{
return "You don't have the permission";
}
}
public function destroy($id)
{
if (Auth::user()->hasRole('administrator')) {
try {
//to get image of the category to be deleted. in Category model, there is function called image
$image = Category::find($id)->thumbnail;
//delete category from database
$res['category'] = Category::destroy($id);
if ($image) {
$file = public_path($image->location) . '/' . $image->file_name;
//test if the image file exists or not
if (File::exists($file)) {
//delete the file from the folder
if (File::delete($file)) {
//delete the image of the category from database;
$res['image'] = $image->delete();
}
}
}
if ($res['category'])
return [1];
else
return [0];
} catch (\Exception $e) {
return [0, $e->getMessage()];
}
}else{
return [0, "You don't have the permission"];
}
}
public function getcategory(){
$categorys = Category::select(['category_id', 'name', 'description'
,'category.image_id','category.created_at','category.updated_at'
,'location','file_name'])
->leftJoin(DB::raw('(select image_id, file_name, location from image) AS temp'),'category.image_id', '=', 'temp.image_id')
;///need to use subquery with DB::raw to avoid ambigous of created_at and updated_at when search
if(Auth::user()->hasRole('administrator')){ //is admin, but need to modify
return Datatables::of($categorys)
->addColumn('action', function ($category) {
$html = '<a href="'.route('category.edit', ['category_id' => $category->category_id]).'" class="btn btn-primary btn-sm"><i class="far fa-edit"></i> Edit</a> ';
$html .= '<a data-id="'.$category->category_id.'" class="btn btn-danger btn-sm category-delete"><i class="far fa-trash-alt"></i></i> Delete</a> ' ;
return $html;
})
->make(true);
}else{
return Datatables::of($categorys)
->addColumn('action', function ($category) {
return "No action";
})->make(true);
}
}
}
<file_sep>/database/migrations/2019_05_12_074705_create_featured_product_table.php
<?php
use Illuminate\Support\Facades\Schema;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Database\Migrations\Migration;
class CreateFeaturedProductTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('featured_product', function (Blueprint $table) {
$table->unsignedInteger('product_id');
$table->date('start_date_time')->nullable();
$table->date('end_date_time')->nullable();
$table->text('status')->nullable();
$table->foreign('product_id')->references('product_id')->on('product'); //on product not seller
$table->dateTime('created_at')->default(DB::raw('CURRENT_TIMESTAMP'));
$table->dateTime('updated_at')->default(DB::raw('CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP'));
//add primary key
$table->primary(['product_id', 'start_date_time','end_date_time'],"primaryindex");
$table->engine = 'InnoDB';
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::drop('featured_product');
}
}
<file_sep>/app/Http/Controllers/Frontend/HomeController.php
<?php
namespace App\Http\Controllers\Frontend;
use App\Http\Controllers\Controller;
use Illuminate\Support\Facades\Auth;
use App\Product;
use App\Category;
use App\Seller;
use App\About;
use PharIo\Manifest\Author;
/**
* Class HomeController.
*/
class HomeController extends Controller
{
/**
* @return \Illuminate\View\View
*/
public function index()
{
$categories = Category::all();
$about = About::first();
if (Auth::check()){
$products = Product::getProductsWithThumbnailCategory(0,0,0,1);
}else{
$products = Product::getProductsWithThumbnailCategory(0,0,0,0);
}
$totalSize = Product::getSize(0,0);
return view('frontend.index', ['categories' => $categories, 'products' => $products , 'about' => $about, 'totalSize' =>$totalSize]);
}
public function shop(){
$sellers = Seller::getSellersWithImage();
$categories = Category::all();
$about = About::first();
$totalSize = Seller::count();
return view('frontend.shop', ['sellers' => $sellers, 'categories' => $categories, 'about' => $about , 'totalSize' => $totalSize]);
//return view('frontend.shop');
}
public function features(){
$categories = Category::all();
$about = About::first();
$totalSize = Product::getSize(0,1);
if(Auth::check()){
$products = Product::getProductsWithThumbnailCategory(0,0,1,1);
}else{
$products = Product::getProductsWithThumbnailCategory(0,0,1,0);
}
return view('frontend.features', ['categories' => $categories, 'products' => $products
, 'about' => $about , 'totalSize' => $totalSize]);
}
public function about(){
$categories = Category::all();
$about = About::first();
if (!$about){
$about = new About();
$about->phone = '012 123 456';
$about->email = '<EMAIL>';
$about->website = 'www.shop.com';
$about->address = '#12, Happy Ave. Phnom Penh Cambodia';
$about->save();
}
return view('frontend.about' , ['about' => $about, 'categories' => $categories]);
}
public function contact(){
$categories = Category::all();
$about = About::first();
return view('frontend.contact',[ 'categories' => $categories, 'about' => $about]);
}
}
<file_sep>/app/Http/Controllers/ProductController.php
<?php
namespace App\Http\Controllers;
use App\About;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\File;
use Illuminate\Support\Facades\Auth;
use App\Product;
use App\Image;
use App\Category;
use App\Post;
use App\Seller;
use App\Like;
use Datatables;
use DB;
class ProductController extends Controller
{
protected $productwithpost;
public function index()
{
return view('scrud.productindex');
}
public function create()
{
$categories = Category::getSelectOptions();
$seller = Seller::where('user_id', Auth::id())->first();
return view('scrud.productcreate', ['categories' => $categories, 'pickup_address' => $seller->address]);
}
public function createwitholdpost($post_id)
{
$categories = Category::getSelectOptions();
$product = Product::where('post_id', '=', $post_id)->first();
return view('scrud.productcreate', ['categories' => $categories
, 'post_id' => $post_id
, 'pickup_time' => $product->pickup_time
, 'pickup_address' => $product->pickup_address]);
}
public function store(Request $request)
{
$product = new Product();
//$product->product_id = $request->get('product_id');
$product->name = $request->get('name');
$product->price = $request->get('price');
$product->description = $request->get('description');
$product->like_number = $request->get('like_number');
$product->status = $request->get('status');
$product->pickup_address = $request->get('pickup_address');
$product->pickup_time = $request->get('pickup_time');
//$product->created_at = $request->get('created_at');
//$product->updated_at = $request->get('updated_at');
//$product->post_id = $request->get('post_id');
//$product->image_id = $request->get('image_id');
$validateData = $request->validate([
'thumbnail_id' => 'required|image|mimes:jpeg,png,jpg,gif,svg|max:2048',
'photos[]' => 'image|mimes:jpeg,png,jpg,gif,svg|max:2048']);
//get file from input
$file = $request->file('thumbnail_id');
$thumbnail = new Image();
$thumbnail->file_name = rand(1111, 9999) . time() . '.' . $file->getClientOriginalExtension();
$thumbnail->location = 'images/thumbnail'; //thumbnail is stored in public/images/thumbnail
try {
$thumbnail->save();
//product the file to it's location on server
$file->move(public_path($thumbnail->location), $thumbnail->file_name);
//thumbnail of product
$product->image_id = $thumbnail->image_id;
if ($request->get('post_id') !== null) {
$product->post_id = $request->get('post_id');
} else {
$user_id = Auth::id();
$seller = Seller::where('user_id', '=', $user_id)->first();
$post = new Post();
$post->seller_id = $seller->seller_id;
$post->save();
$product->post_id = $post->post_id;
}
//add seller is missing
$product->save();
//test if user has upload other photos or not
if ($request->hasFile('photos')) {
//get the array of photos
$photos = $request->file('photos');
foreach ($photos as $key => $file) {
$photo = new Image();
$photo->file_name = rand(1111, 9999) . time() . '.' . $file->getClientOriginalExtension();
//photos are stored on server in folder public/images/photos
$photo->location = 'images/photos';
//photo belongs to product
$photo->product_id = $product->product_id; //not (id) product_id
$photo->save();
$file->move(public_path($photo->location), $photo->file_name);
}
}
$category = $request->get('category_id');
if (sizeof($category) > 0) {
$product->category()->attach($category);
}
//dd($category);
if ($request->get('add_more') == 1) {
return redirect()->route('product.create.with.oldpost', ['post_id' => $product->post_id])->withFlashSuccess('Product is added. You wish you add more product in the same post. We suggest the same pickup time and date');
}
return redirect()->route('product.index')->withFlashSuccess('Product is added');
} catch (\Exception $e) {
return redirect()
->back()
->withInput($request->all())
->withFlashDanger("Product can't be added. " . $e->getMessage());
}
}
public function show($id)
{
echo 'showlalal' . $id;
}
public function edit($id)
{
$product = Product::with('thumbnail')->with('photo')->with('post')->find($id);
$post = $product->post;
$seller = Seller::find($post->seller_id);
$categories = Category::getSelectOptions();
if (Auth::user()->hasRole('administrator')) {
return view('scrud.productedit', ['categories' => $categories, 'product' => $product]);
} elseif ($seller->user_id == Auth::id()) {
return view('scrud.productedit', ['categories' => $categories, 'product' => $product]);
} else {
return redirect()->back()->withFlashDanger("You don't have the permission");
}
}
public function update(Request $request, $id)
{
$product = Product::with('thumbnail')->with('photo')->with('post')->find($id);
$permit = false;
if (Auth::user()->hasRole('administrator')) {
$permit = true;
} else {
$post = $product->post;
$seller = Seller::find($post->seller_id);
// $user = Auth::id();
//$seller = Seller::where('user_id', $user)->first();
if ($seller->user_id == Auth::id()) {
$permit = true;
} else {
return redirect()
->back()
->withFlashDanger("You dont have the permission ");
}
}
if ($permit) {
//$product = Product::find($id);
//$product->product_id = $request->get('product_id');
$product->name = $request->get('name');
$product->price = $request->get('price');
$product->description = $request->get('description');
//$product->like_number = $request->get('like_number');
$product->status = $request->get('status');
$product->pickup_address = $request->get('pickup_address');
$product->pickup_time = $request->get('pickup_time');
//$product->created_at = $request->get('created_at');
//$product->updated_at = $request->get('updated_at');
//$product->post_id = $request->get('post_id');
//$product->image_id = $request->get('image_id');
$validateData = $request->validate([
'thumbnail_id' => 'image|mimes:jpeg,png,jpg,gif,svg|max:2048'
, 'photos[]' => 'image|mimes:jpeg,png,jpg,gif,svg|max:2048']);
try {
// test if thumbnail is updated or not
if ($request->hasFile('thumbnail_id')) {
$file = $request->file('thumbnail_id');
$thumbnail = new Image();
$thumbnail->file_name = rand(1111, 9999) . time() . '.' . $file->getClientOriginalExtension();
$thumbnail->location = 'images/thumbnail';
$file->move(public_path($thumbnail->location), $thumbnail->file_name);
$thumbnail->save();//save new thumbnail
$old_thumbnail = $product->thumbnail; // Keep the old thumbnail for removing if it exists
$product->image_id = $thumbnail->image_id; //change the thumbnail to the new one
}
$product->save(); //save the update of product
if (isset($old_thumbnail)) {
//remove old thumbnail from harddisk
$file = public_path($old_thumbnail->location) . '/' . $old_thumbnail->file_name;
if (File::exists($file)) {
File::delete($file);
}
$old_thumbnail->delete(); //delete the old thumbnail if user add a new one
}
$db_old_photos = $product->photo;//get old photos from db
if ($db_old_photos) {// if there is any old photos in db
$old_photos = $request->get('old_photos'); //get the list of old photos after use update
// print_r($old_photos);
foreach ($db_old_photos as $db_old_photo) {
//test if user has deleted all old photos, we remove it from db and hard disk
//or test if some old photos are deleted by user, we remove it form db and hard disk
if (!$old_photos or ($old_photos && !in_array($db_old_photo->image_id, $old_photos))) {
if ($db_old_photo->delete()) {
//remove old thumbnail from harddisk
$file = public_path($db_old_photo->location) . '/' . $db_old_photo->file_name;
if (File::exists($file)) {
File::delete($file);
}
}
}
}
}
//test if user has upload other photos or not
if ($request->hasFile('photos')) {
//get the array of photos
$photos = $request->file('photos');
foreach ($photos as $file) {
$photo = new Image();
$photo->file_name = rand(1111, 9999) . time() . '.' . $file->getClientOriginalExtension();
//photos are stored on server in folder public/images/photos
$photo->location = 'images\photos';
//photo belongs to product
$photo->product_id = $request->get('product_id');
$photo->save();
$file->move(public_path($photo->location), $photo->file_name);
}
}
$category = $request->get('category_id');
$old_category = $product->category;
$array_old_category = $old_category->pluck('category_id')->toArray();
print_r($array_old_category);
foreach ($old_category as $ele){
if (!in_array ( $ele->category_id, $category )){
$product->category()->detach($ele->category_id);
}
}
foreach ($category as $ele){
if (!in_array ( $ele, $array_old_category )){
$product->category()->attach($ele);
}
}
return redirect()->route('product.index')->withFlashSuccess('product is updated');
} catch (\Exception $e) {
return redirect()
->back()
->withInput($request->all())
->withFlashDanger("Product can't be updated. " . $e->getMessage());
}
}
}
public function destroy($id){
$product = Product::with('thumbnail')->with('photo')->with('post')->find($id);
$permit = false;
if (Auth::user()->hasRole('administrator')) {
$permit = false;
} else {
$post = $product->post;
$seller = Seller::find($post->seller_id);
// $user = Auth::id();
//$seller = Seller::where('user_id', $user)->first();
if ($seller->user_id == Auth::id()) {
$permit = true;
} else {
return redirect()
->back()
->withFlashDanger("You dont have the permission ");
}
}
if ($permit) {
try {
//to get the array of photos of the product
$product = Product::with('photo')->with('thumbnail')->find($id);
$photos = $product->photo;
$res['photos'] = true;
if ($photos) {
foreach ($photos as $photo) {
$file = public_path($photo->location) . '\\' . $photo->file_name;
if (File::exists($file)) {
if (File::delete($file)) {//delete the file from the folder
$res['photos'] = $res['photos'] && $photo->delete(); //delete the file from database
}
}
}
}
//to get thumbnail of the product to be deleted. in product model, there is function called thumbnail
$thumbnail = $product->thumbnail;
$post = $product->post;
//delete product from database
$res['product'] = Product::destroy($id);
$otherproduct = $post->product;
if (sizeof($otherproduct) == 0) {
$post->delete();
}
if ($thumbnail) {
$file = $file = public_path($thumbnail->location) . '\\' . $thumbnail->file_name;
//test if the thumbnail file exists or not
if (File::exists($file)) {
//delete the file from the folder
if (File::delete($file)) {
//delete the thumbnail of the product from database;
$res['thumbnail'] = $thumbnail->delete();
}
}
}
if ($res['product'])
return [1];
else
return [0];
} catch (\Exception $e) {
return [0, $e->getMessage()];
}
}
}
public function getproduct(){
if(Auth::user()->hasRole('administrator')){ //is admin, but need to modify
$products = Product::select(['product.product_id', 'product.name'/*DB::raw('product.name as pname')*/, 'price'
, 'description','like_number','status','pickup_address','pickup_time','created_at'
,'updated_at', 'file_name', 'location'])
->leftJoin(DB::raw('(select image_id, file_name, location from image) as temp'),'product.image_id', '=', 'temp.image_id')
->with('category')
;
}else{
$user = Auth::id();
$seller = Seller::where('user_id',$user)->first();
$products = Product::select(['product.product_id', 'product.name'/*DB::raw('product.name as pname')*/, 'price'
, 'description','like_number','status','pickup_address','pickup_time','created_at'
,'updated_at', 'file_name', 'location', 'temp1.seller_id'])
->leftJoin(DB::raw('(select image_id, file_name, location from image) as temp'),'product.image_id', '=', 'temp.image_id')
->leftJoin(DB::raw('(select seller.seller_id, post.post_id from seller join post on post.seller_id = seller.seller_id) as temp1')
,'product.post_id', '=', 'temp1.post_id')
->with('category')
->where('temp1.seller_id' , $seller->seller_id)
;
return Datatables::of($products)
->addColumn('action', function ($product) {
$html = '<a href="'.route('product.edit', ['id' => $product->product_id]).'" class="btn btn-primary btn-sm"><i class="far fa-edit"></i></a> ';
$html .= '<a data-id="'.$product->product_id.'" class="btn btn-danger btn-sm product-delete"><i class="far fa-trash-alt"></i></a> ' ;
return $html;
})
->make(true);
}
return Datatables::of($products)
->addColumn('action', function ($product) {
$html = '<a href="'.route('product.edit', ['id' => $product->product_id]).'" class="btn btn-primary btn-sm"><i class="far fa-edit"></i></a> ';
$html .= '<a data-id="'.$product->product_id.'" class="btn btn-danger btn-sm product-delete"><i class="far fa-trash-alt"></i></a> ' ;
$html .= '<a data-id="'.$product->product_id.'" class="btn btn-info btn-sm product-featured" data-toggle="modal" data-target="#featured_product_modal"><i class="fas fa-cog"></i></a>' ;
return $html;
})
->make(true);
}
public function getphotos(Request $request){
$product_id = $request->get('product_id');
//get the list of photos of product using relationship defined in model
$photos = Product::find($product_id)->photo;
$thumbnail = Product::find($product_id)->thumbnail;
if($thumbnail){
$photos->push($thumbnail);
}
if (sizeof($photos) > 0 || $thumbnail){
$html = "";
$source = "";
$eleclass = "";
$i=0;
foreach ($photos as $photo) {
//get url of each photo
$source = asset(str_replace('\\','/',$photo->location)) . "/" . $photo->file_name;
if ($i==0){
//set class start to the first photo, so we can use js to click it
$eleclass = "class='start'";
$i = 1;
}
else
$eleclass = "";
//html code for each photo html element
$html .= "<a href='" . $source . "' " . $eleclass . " ><img src='" . $source ."' height='40' width='40' ></a>";
}
//list of photos must be in the dive with id lightgallery, so in view we can apply the lightgallery library on it
$html = "<div id='lightgallery'>" . $html . "</div>";
return [1, $html];
}else
return [0];
}
//add access control
public function getactivefeatured(Request $request){
$product_id = $request->get('product_id');
$product = Product::with('thumbnail')->with('photo')->with('post')->find($product_id);
$permit = false;
if (Auth::user()->hasRole('administrator')) {
$permit = true;
}
if ($permit) {
$featured_product = Product::getactivefeatured($product_id);
if (sizeof($featured_product) > 0) {
return [1, $featured_product];
} else {
return [0];
}
}else{
return [2,"You don't have the permission. "];
}
}
//add access control
public function savefeatured(Request $request)
{
$product_id = $request->get('product_id');
$product = Product::with('thumbnail')->with('photo')->with('post')->find($product_id);
$permit = false;
if (Auth::user()->hasRole('administrator')) {
$permit = true;
}
if ($permit) {
$start_date = $request->get('start_date');
$end_date = $request->get('end_date');
$featured_product = Product::getactivefeatured($product_id);
if ($end_date == null) {
$end_date = '9999-01-01';
}
if (sizeof($featured_product) == 1) {
try {
Product::updatefeatured($product_id, $start_date, $end_date);
return [1,1,$product_id];
} catch (\Exception $e) {
return [0, $e->getMessage(),$product_id];
}
} else {
try {
Product::savefeatured($product_id, $start_date, $end_date);
return [1,1,$product_id];
} catch (\Exception $e) {
return [0, $e->getMessage(),$product_id];
}
}
}else{
return [2,"You don't have the permission. ", $product_id];
}
}
public function getproductmore(Request $request){
if(Auth::check()){
$products = Product::getProductsWithThumbnailCategory($request->get('offset'), $request->get('seller')
, $request->get('features'), 1);
}else{
$products = Product::getProductsWithThumbnailCategory($request->get('offset'), $request->get('seller')
,$request->get('features'),0);
}
if(sizeof($products) > 0){
$items = array();
foreach ($products as $product){
$category = "";
$category_name = "";
$categories = $product->category;
foreach ($categories as $ele){
$category .= str_replace(' ','-',$ele->name). " ";
$category_name .= $ele->name. ", ";
}
$category_name = substr($category_name,0,strlen($category_name )-2);
$html = "";
$html .= <<<eot
<div class="col-sm-6 col-md-4 col-lg-3 p-b-35 isotope-item $category" data-product_id="$product->product_id">
<!-- Block2 -->
<div class="block2" style="height:100%">
<div class="block2-pic hov-img0" >
eot;
if($product->file_name){
$location = asset($product->location);
$html .= <<<eot
<img src="$location/$product->file_name" alt="IMG-PRODUCT">
eot;
}else{
$location = asset('images/thumbnail');
$html .= <<<eot
<img src="$location/default.png" alt="IMG-PRODUCT">
eot;
}
$location = asset('cozastore');
$html .= <<<eot
<a href="javascript:void(0);" class="block2-btn flex-c-m stext-103 cl2 size-102 bg0 bor2 hov-btn1 p-lr-15 trans-04 js-show-modal1" data-product_id="$product->product_id">
Quick View
</a>
</div>
<div class="block2-txt flex-w flex-t p-t-14">
<div class="block2-txt-child1 flex-col-l ">
<span class="stext-105 cl3">
eot;
$active_featured_product = Product::getactivefeatured($product->product_id);
if (sizeof($active_featured_product) == 1){
$html .= <<<eot
<b class='pname'>$product->name</b>
<sup style="color:white;background-color:red;border-radius: 10px;"> Hot </sup>
eot;
}else{
$html .= <<<eot
<b class='pname'>$product->name</b>
eot;
}
$html .= <<<eot
</span>
<span class="stext-105 cl3 price">
$product->price
</span>
<span class="stext-105 cl3 category">
$category_name
</span>
</div>
<div class="block2-txt-child2 flex-r p-t-3">
eot;
$like = $product->like;
$location = asset('cozastore');
$number_like = sizeof($like);
if($number_like > 0){
$user_id = optional(auth()->user())->id;
$number_like = sizeof($like);
foreach($like as $ele){
if($user_id && $user_id == $ele->user_id){
$html .= <<<eot
<span class="number-like">$number_like</span>
<a href="javascript:void(0);" class="btn-addwish-b2 dis-block pos-relative js-addwish-b2 js-addedwish-b2" data-product_id="$product->product_id">
<img class="icon-heart1 dis-block trans-04" src="$location/images/icons/icon-heart-01.png" alt="ICON">
<img class="icon-heart2 dis-block trans-04 ab-t-l" src="$location/images/icons/icon-heart-02.png" alt="ICON">
</a>
eot;
}else{
$html .= <<<eot
<span class="number-like">$number_like</span>
<a href="javascript:void(0);" class="btn-addwish-b2 dis-block pos-relative js-addwish-b2" data-product_id="$product->product_id">
<img class="icon-heart1 dis-block trans-04" src="$location/images/icons/icon-heart-01.png" alt="ICON">
<img class="icon-heart2 dis-block trans-04 ab-t-l" src="$location/images/icons/icon-heart-02.png" alt="ICON">
</a>
eot;
}
}
}else{
$html .= <<<eot
<span class="number-like">$number_like</span>
<a href="javascript:void(0);" class="btn-addwish-b2 dis-block pos-relative js-addwish-b2" data-product_id="$product->product_id">
<img class="icon-heart1 dis-block trans-04" src="$location/images/icons/icon-heart-01.png" alt="ICON">
<img class="icon-heart2 dis-block trans-04 ab-t-l" src="$location/images/icons/icon-heart-02.png" alt="ICON">
</a>
eot;
}
$html .= <<<eot
</div>
</div>
</div>
</div>
eot;
$items[] = $html;
}
//return [1,$html];
$totalSize = Product::getSize( $request->get('seller'),$request->get('features'));
return [1, $totalSize,$items];
}
else{
$totalSize = Product::getSize( $request->get('seller'),$request->get('features'));
return [0, $totalSize];
}
}
public function getproductdetail(Request $request){
$product = Product::with('photo')->with('thumbnail')->with('category')->find($request->get('product_id'));
$post = Post::where('post_id',$product->post_id)->first();
$seller = Seller::where('seller_id',$post->seller_id)->first();
if(isset($product->thumbnail)){
$location = str_replace('\\','/',$product->thumbnail->location);
$product->thumbnail->location = $location;
}else{
$product->thumbnail_id = asset('images/thumbnail').'/default.png';
}
if(isset($product->photos)){
$size = sizeof($product->photo);
for($i = 0 ; $i < $size; $i ++){
$location = asset(str_replace('\\','/',$product->photos[$i]->location));
$product->photos[$i]->location = $location;
}
}
return [1,$product, $seller];
}
public function likeUnlike(Request $request)
{
if(Auth::check()){
$product_id = $request->get('product_id');
$operation = $request->get('operation');
$product = Product::find($product_id);
if (!$product) {
return 0;
}
$user_id = Auth::id();
if($operation == 'like'){
$like = new Like();
$like->user_id = $user_id;
$like->product_id = $product_id;
$like->save();
$product = Product::find($product_id);
$product->like_number = $product->like()->count();
$product->save();
return 1;
}elseif ($operation == 'unlike'){
$like = Like::where('product_id',$product_id)
->where('user_id',$user_id)->first();
if($like){
DB::table('like')
->where('product_id',$product_id)
->where('user_id',$user_id)
->delete();
$product = Product::find($product_id);
$product->like_number = $product->like()->count();
$product->save();
}
return 1;
}
}else{
return 2;
}
}
public function showProductByShop($seller_id){
$categories = Category::all();
$about = About::first();
$seller = Seller::find($seller_id);
$totalSize = Product::getSize($seller_id,0);
if (Auth::check()){
$products = Product::getProductsWithThumbnailCategory(0,$seller_id,0,1);
}else{
$products = Product::getProductsWithThumbnailCategory(0,$seller_id,0,0);
}
return view('frontend.shopproduct', ['categories' => $categories, 'products' => $products
, 'about' => $about , 'seller' =>$seller, 'totalSize' =>$totalSize]);
}
public function showProductDetail($product_id){
$categories = Category::all();
$about = About::first();
$request = new Request();
$request->setMethod('get');
$request->request->add(['product_id' => $product_id]);
$data = $this->getproductdetail($request);
return view('frontend.productdetail',['categories' => $categories
, 'about' => $about, 'product' => $data[1], 'seller'=>$data[2]]);
}
}
<file_sep>/routes/breadcrumbs/backend/product.php
<?php
Breadcrumbs::for('product.index', function ($trail) {
$trail->push( 'List Product', route('product.index'));
});
Breadcrumbs::for('product.create', function ($trail) {
$trail->parent('product.index');
$trail->push( 'Create product', route('product.create'));
});
Breadcrumbs::for('product.create.with.oldpost', function ($trail) {
$trail->parent('product.index');
$trail->push( 'Create product', route('product.create'));
});
Breadcrumbs::for('product.edit', function ($trail,$id) {
$trail->parent('product.index');
$trail->push( 'Create product', route('product.edit',$id));
});
<file_sep>/app/Http/Controllers/MessageController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Auth;
use Datatables;
use App\Message;
class MessageController extends Controller
{
public function index() {
if(Auth::user()->hasRole('administrator')){ //is admin, but need to modify
return view('scrud.messageindex');
}else{
return redirect()->back()->withFlashDanger("You don't have the permission");
}
}
public function getmessage(){
// to be change
$messages = Message::select(['message_id', 'full_name','email','phone', 'message','status','message.created_at','message.updated_at'])->get();
// if(Auth::user()->hasRole('administrator')){ //is admin, but need to modify
//return Datatables::of($messages)
return Datatables::of($messages)
->addColumn('action', function ($message) {
$html = '<a href="'.route('message.markread', ['id' => $message->message_id]).'" class="btn btn-primary btn-sm"><i class="far fa-envelope-open"></i></a>';
return $html;
})
->make(true);
// }
}
public function markread(Request $request){
$message_id = $request->get('id');
$message = Message::find($message_id);
$message->status = 1;
$message->save();
return redirect()->back();
}
public function getUnread(){
if (Auth::user()->hasRole('administrator'))
return Message::where('status',0)->count();
else
return 0;
}
}
<file_sep>/app/post.php
<?php
namespace App;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Support\Facades\App;
class Post extends Model
{
public $primaryKey = 'post_id';
public $table = 'post';
public function product()//not function command
{
return $this->hasMany('App\Product','post_id','post_id');
}
public function seller(){ //create seller function
return $this->belongsTo('App\Seller','seller_id','seller_id');
}
}
<file_sep>/app/product.php
<?php
namespace App;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Support\Facades\DB;
class Product extends Model
{
//
protected $table ='product';
protected $primaryKey = 'product_id';
public static function getProduct($product_id)
{
$sql = "select * from product";
return DB::select($sql);
}
public function post()
{
return $this->belongsTo('App\Post','post_id','post_id');
}
public function category()
{
return $this->belongsToMany('App\Category','product_category', 'product_id','category_id'); //belong to many category
}
public function thumbnail()
{
return $this->belongsTo('App\Image','image_id','image_id');
}
public function photo()
{
return $this->hasMany('App\Image','product_id','product_id');
}
public function featured_product()//add function featured_product
{
return $this->hasMany('App\FeaturedProduct','product_id','product_id');
}
public static function getactivefeatured($product_id){
$sql = <<<EOT
select date(start_date_time) as start_date, date(end_date_time) as end_date
from featured_product
where product_id = $product_id and (date(end_date_time) >= curdate())
order by updated_at desc
limit 0, 1;
EOT;
return DB::select($sql);
}
public static function savefeatured($product_id, $start_date, $end_date){
$sql = <<<EOT
insert into featured_product(product_id, start_date_time, end_date_time)
values($product_id, '$start_date', '$end_date');
EOT;
return DB::insert($sql);
}
public static function updatefeatured($product_id, $start_date, $end_date){
$old_featured = Product::getactivefeatured($product_id);
$old_featured = $old_featured[0];
$sql = <<<EOT
update featured_product set
start_date_time = '$start_date',
end_date_time = '$end_date'
where product_id = $product_id
and date(start_date_time) = '$old_featured->start_date'
and date(end_date_time) = '$old_featured->end_date';
EOT;
return DB::update($sql);
}
public static function getProductsWithThumbnailCategory($offset=0,$seller_id=0, $features = 0, $like =0){
$products = Product::select(['product.product_id', 'product.name', 'price'
, 'description','like_number','status','pickup_address','pickup_time','created_at'
,'updated_at', 'file_name', 'location'])
->leftJoin(DB::raw('(select image_id, file_name, location from image) as temp')
,'product.image_id', '=', 'temp.image_id')
->where('status','Available')
->with('category');
if ($seller_id != 0){
$products = $products->leftJoin(DB::raw('(select post_id, seller_id from post) as temp1')
,'product.post_id', '=', 'temp1.post_id')
->where('temp1.seller_id',$seller_id);
}
if($like ==1){
$products = $products->with('like');
}
if($features == 1){
$products = $products->join(DB::raw('(select product_id from featured_product where (date(end_date_time) >= curdate() and date(start_date_time) <= curdate()) group by product_id ) as temp2')
, 'temp2.product_id','product.product_id');
}
$products = $products->offset($offset)
->take(4)
->orderBy('product.product_id','desc')
->get();
return $products;
}
public function like()
{
return $this->hasMany('App\Like','product_id', 'product_id'); //belong to many category
}
public static function getSize($seller_id, $features){
$products = Product::where('status','Available');
if ($seller_id != 0){
$products = $products->leftJoin(DB::raw('(select post_id, seller_id from post) as temp1')
,'product.post_id', '=', 'temp1.post_id')
->where('temp1.seller_id',$seller_id);
}
if($features == 1){
$products = $products->join(DB::raw('(select product_id from featured_product where (date(end_date_time) >= curdate() and date(start_date_time) <= curdate()) group by product_id ) as temp2')
, 'temp2.product_id','product.product_id');
}
$size = $products->count();
return $size;
}
}
<file_sep>/public/cozastore/js/main.js
(function ($) {
"use strict";
/*[ Load page ]
===========================================================*/
$(".animsition").animsition({
inClass: 'fade-in',
outClass: 'fade-out',
inDuration: 1500,
outDuration: 800,
linkElement: '.animsition-link',
loading: true,
loadingParentElement: 'html',
loadingClass: 'animsition-loading-1',
loadingInner: '<div class="loader05"></div>',
timeout: false,
timeoutCountdown: 5000,
onLoadEvent: true,
browser: [ 'animation-duration', '-webkit-animation-duration'],
overlay : false,
overlayClass : 'animsition-overlay-slide',
overlayParentElement : 'html',
transition: function(url){ window.location.href = url; }
});
/*[ Back to top ]
===========================================================*/
var windowH = $(window).height()/2;
$(window).on('scroll',function(){
if ($(this).scrollTop() > windowH) {
$("#myBtn").css('display','flex');
} else {
$("#myBtn").css('display','none');
}
$('.isotope-grid').isotope('layout');
});
$('#myBtn').on("click", function(){
$('html, body').animate({scrollTop: 0}, 300);
});
/*==================================================================
[ Fixed Header ]*/
var headerDesktop = $('.container-menu-desktop');
var wrapMenu = $('.wrap-menu-desktop');
if($('.top-bar').length > 0) {
var posWrapHeader = $('.top-bar').height();
}
else {
var posWrapHeader = 0;
}
if($(window).scrollTop() > posWrapHeader) {
$(headerDesktop).addClass('fix-menu-desktop');
$(wrapMenu).css('top',0);
}
else {
$(headerDesktop).removeClass('fix-menu-desktop');
$(wrapMenu).css('top',posWrapHeader - $(this).scrollTop());
}
$(window).on('scroll',function(){
if($(this).scrollTop() > posWrapHeader) {
$(headerDesktop).addClass('fix-menu-desktop');
$(wrapMenu).css('top',0);
}
else {
$(headerDesktop).removeClass('fix-menu-desktop');
$(wrapMenu).css('top',posWrapHeader - $(this).scrollTop());
}
});
/*==================================================================
[ Menu mobile ]*/
$('.btn-show-menu-mobile').on('click', function(){
$(this).toggleClass('is-active');
$('.menu-mobile').slideToggle();
});
var arrowMainMenu = $('.arrow-main-menu-m');
for(var i=0; i<arrowMainMenu.length; i++){
$(arrowMainMenu[i]).on('click', function(){
$(this).parent().find('.sub-menu-m').slideToggle();
$(this).toggleClass('turn-arrow-main-menu-m');
})
}
$(window).resize(function(){
if($(window).width() >= 992){
if($('.menu-mobile').css('display') == 'block') {
$('.menu-mobile').css('display','none');
$('.btn-show-menu-mobile').toggleClass('is-active');
}
$('.sub-menu-m').each(function(){
if($(this).css('display') == 'block') { console.log('hello');
$(this).css('display','none');
$(arrowMainMenu).removeClass('turn-arrow-main-menu-m');
}
});
}
});
/*==================================================================
[ Show / hide modal search ]*/
$('.js-show-modal-search').on('click', function(){
$('.modal-search-header').addClass('show-modal-search');
$(this).css('opacity','0');
});
$('.js-hide-modal-search').on('click', function(){
$('.modal-search-header').removeClass('show-modal-search');
$('.js-show-modal-search').css('opacity','1');
});
$('.container-search-header').on('click', function(e){
e.stopPropagation();
});
/*==================================================================
[ Isotope ] */
var $topeContainer = $('.isotope-grid');
var $filter = $('.filter-tope-group');
// filter items on button click
/*$filter.each(function () {
$filter.on('click', 'button', function () {
var filterValue = $(this).attr('data-filter');
$topeContainer.isotope({filter: filterValue});
});
});
*/
/////////////////////edited by vanna
function perform_filter($this){
var price = Number($this.find('.price').text());
var filter_by = $('.filter-price').val();
var active_category = $('.how-active1').data('filter').substring(1);
var filter_by_name = $('#search_name').val().trim().toLowerCase().split(' ');
var product_name = $this.find('.pname').text().toLowerCase();
var is_in_price;
var is_in_category;
var is_in_name = false;
var i;
// alert('imaher');
//$('.testoutput').append('<p>'+active_director+'</p>'+'<p>'+filter_by+'</p>');
// selected price
// console.log(filter_by_name);
// console.log(product_name);
is_in_price = ( filter_by === 'all'
|| (filter_by === 'after200' && price >= 200)
|| (price >= (Number(filter_by) - 50) && price <= Number(filter_by) )
);
is_in_category = ( active_category === ''
|| $this.hasClass(active_category)
);
if (filter_by_name.length == 0)
is_in_name = true;
else {
for (i =0; i < filter_by_name.length ; i++){
if (product_name.indexOf(filter_by_name[i] ) != -1){
is_in_name = true;
break;
}
}
}
return is_in_name && is_in_price && is_in_category;
// if (isNaN(filter_by)){
// if (filter_by == 'all'){
// if ( active_category === ''){
// return !(product_name.indexOf(filter_by_name) === -1);
// }
// else{
// return ($this.hasClass(active_category) && !(product_name.indexOf(filter_by_name) === -1 ));
// }
// }else{
// if ( active_category === '')
// return ((price >= 200 ) && !(product_name.indexOf(filter_by_name) === -1));
// else
// return ( (price >= 200 ) && $this.hasClass(active_category) && !(product_name.indexOf(filter_by_name) === -1)) ;
// }
// }else{
// filter_by = Number(filter_by);
// if (price >= (filter_by - 50) && price <= filter_by){
// if ( active_category === '')
// return true && !(product_name.indexOf(filter_by_name) === -1);
// else
// return $this.hasClass(active_category) && !(product_name.indexOf(filter_by_name) === -1);
// }
// else
// return false;
// }
}
$filter.each(function () {
$filter.on('click', 'button', function () {
//var filterValue = $(this).attr('data-filter');
$topeContainer.isotope({
filter: function(){
return perform_filter($(this));
}
});
updateSize();
});
})
// init Isotope
$(window).on('load', function () {
var $grid = $topeContainer.each(function () {
$(this).isotope({
itemSelector: '.isotope-item',
layoutMode: 'fitRows',
percentPosition: true,
animationEngine : 'best-available',
masonry: {
columnWidth: '.isotope-item'
}
});
});
});
var isotopeButton = $('.filter-tope-group button');
$(isotopeButton).each(function(){
$(this).on('click', function(){
for(var i=0; i<isotopeButton.length; i++) {
$(isotopeButton[i]).removeClass('how-active1');
}
$(this).addClass('how-active1');
});
});
/*==================================================================
[ Filter / Search product ]*/
$('.js-show-filter').on('click',function(){
$(this).toggleClass('show-filter');
$('.panel-filter').slideToggle(400);
if($('.js-show-search').hasClass('show-search')) {
$('.js-show-search').removeClass('show-search');
$('.panel-search').slideUp(400);
}
});
$('.js-show-search').on('click',function(){
$(this).toggleClass('show-search');
$('.panel-search').slideToggle(400);
if($('.js-show-filter').hasClass('show-filter')) {
$('.js-show-filter').removeClass('show-filter');
$('.panel-filter').slideUp(400);
}
});
/*==================================================================
[ Cart ]*/
$('.js-show-cart').on('click',function(){
$('.js-panel-cart').addClass('show-header-cart');
});
$('.js-hide-cart').on('click',function(){
$('.js-panel-cart').removeClass('show-header-cart');
});
/*==================================================================
[ Cart ]*/
$('.js-show-sidebar').on('click',function(){
$('.js-sidebar').addClass('show-sidebar');
});
$('.js-hide-sidebar').on('click',function(){
$('.js-sidebar').removeClass('show-sidebar');
});
/*==================================================================
[ +/- num product ]*/
$('.btn-num-product-down').on('click', function(){
var numProduct = Number($(this).next().val());
if(numProduct > 0) $(this).next().val(numProduct - 1);
});
$('.btn-num-product-up').on('click', function(){
var numProduct = Number($(this).prev().val());
$(this).prev().val(numProduct + 1);
});
/*==================================================================
[ Rating ]*/
$('.wrap-rating').each(function(){
var item = $(this).find('.item-rating');
var rated = -1;
var input = $(this).find('input');
$(input).val(0);
$(item).on('mouseenter', function(){
var index = item.index(this);
var i = 0;
for(i=0; i<=index; i++) {
$(item[i]).removeClass('zmdi-star-outline');
$(item[i]).addClass('zmdi-star');
}
for(var j=i; j<item.length; j++) {
$(item[j]).addClass('zmdi-star-outline');
$(item[j]).removeClass('zmdi-star');
}
});
$(item).on('click', function(){
var index = item.index(this);
rated = index;
$(input).val(index+1);
});
$(this).on('mouseleave', function(){
var i = 0;
for(i=0; i<=rated; i++) {
$(item[i]).removeClass('zmdi-star-outline');
$(item[i]).addClass('zmdi-star');
}
for(var j=i; j<item.length; j++) {
$(item[j]).addClass('zmdi-star-outline');
$(item[j]).removeClass('zmdi-star');
}
});
});
/*==================================================================
[ Show modal1 ]*/
/*
$('.js-show-modal1').on('click',function(e){
e.preventDefault();
$('.js-modal1').addClass('show-modal1');
});
$('.js-hide-modal1').on('click',function(){
$('.js-modal1').removeClass('show-modal1');
});
*/
//added by vanna
// Quick View
$(document).off('click', '.js-show-modal1');
$(document).on('click', '.js-show-modal1',function(e){
$('.js-modal1').addClass('show-modal1');
$.ajax({
type:"GET",
url:window.location.protocol +'//'+window.location.host+"/admin/product/getproductdetail",
data:{ product_id:$(this).data('product_id') } ,
success: function (data) {
//console.log(data);
if(data[0] == 1){
var gl_container = $('.gallery-lb');
var text_container = $('.detail-text');
var product = data[1];
var location;
var html = "";
var size;
var i;
var temp;
var category_name;
var category;
var seller = data[2];
$('.detail-text').empty();
if (product['photo'].length > 0){
//alert('hter');
size = product['photo'].length;
for (i = 0; i< size ; i++){
/*
location = movie['photos'][i]['location']+'\\'+movie['photos'][i]['file_name'];
html = html + '<a class="flex-c-m size-108 how-pos1 bor0 fs-16 cl10 bg0 hov-btn3 trans-04" href="'
+location+'">';
html = html + '<i class="fa fa-expand"></i></a>';
*/
location = '/'+product['photo'][i]['location']+'/'+product['photo'][i]['file_name'];
html = html + '<div class="item-slick3" data-thumb="'+location+'">';
html = html + '<div class="wrap-pic-w pos-relative">';
html = html + '<img src="'+location+'" alt="IMG-PRODUCT">';
html = html + '<a class="flex-c-m size-108 how-pos1 bor0 fs-16 cl10 bg0 hov-btn3 trans-04" href="'
+location+'">';
html = html + '<i class="fa fa-expand"></i></a></div></div>';
}
gl_container.prepend(html);
html = "";
}
if (product['thumbnail'] !== null){
location ='/'+ product['thumbnail']['location']+'/'+product['thumbnail']['file_name'];
}else{
location ='/'+product['thumbnail_id'];
}
html = '<div class="item-slick3" data-thumb="'+location+'">';
html = html + '<div class="wrap-pic-w pos-relative">';
html = html + '<img src="'+location+'" alt="IMG-PRODUCT">';
html = html + '<a class="flex-c-m size-108 how-pos1 bor0 fs-16 cl10 bg0 hov-btn3 trans-04" href="'
+location+'">';
html = html + '<i class="fa fa-expand"></i></a></div></div>';
/* html = html + '<a class="flex-c-m size-108 how-pos1 bor0 fs-16 cl10 bg0 hov-btn3 trans-04" href="'
+location+'">';
html = html + '<i class="fa fa-expand"></i></a>';
*/
gl_container.prepend(html);
html = "";
category_name = "";
category = product['category'];
//alert(category);
for (i = 0 ; i < category.length; i++){
category_name = category_name + category[i].name + ", ";
}
category_name = category_name.substring(0, category_name.length - 2);
html = '<h4 class="mtext-105 cl2 js-name-detail p-b-14">'+product.name+'</h4>';
html = html + '<span class="mtext-106 cl2">Price : '+product.price+'</span>';
html = html + '<p class="stext-102 cl3 p-t-23">Description : '+product.description+'</p>';
html = html + '<p class="stext-102 cl3 p-t-23">Availability : '+product.status+'</p>';
html = html + '<p class="stext-102 cl3 p-t-23">Like : '+product.like_number+'</p>';
html = html + '<p class="stext-102 cl3 p-t-23"><b>Category : </b>'
+category_name+'</p>';
html = html
+'<p class="stext-102 cl3 p-t-23">'
+ '<b>Seller : </b><a href="/shop/'+seller.seller_id+'">'
+seller.name
+'</a></p>'
+'<p class="stext-102 cl3 p-t-23">'
+ '<b>Address : </b>'
+seller.address
+'</p>'
+'<p class="stext-102 cl3 p-t-23">'
+ '<b>Email : </b>'
+seller.email
+'</p>'
+'<p class="stext-102 cl3 p-t-23">'
+ '<b>Phone : </b>'
+seller.phone
+'</p>'
+'<p class="stext-102 cl3 p-t-23">'
+ '<b>Message_Account : </b>'
+seller.message_account
+'</p>'
+ '<p class="stext-102 cl3 p-t-23">'
+ '<b>Type : </b>'
+seller.type
+'</p>';
html = html + '<div class="flex-w flex-m p-l-100 p-t-40 respon7 facebookshare"></div>'
text_container.prepend(html);
temp = encodeURIComponent(window.location.protocol+'//'
+ window.location.hostname+(window.location.port ? ':'+window.location.port: '')
+ '/product/' + product['product_id'] );
html = '<iframe src="https://www.facebook.com/plugins/share_button.php?href='+temp+'&layout=button&size=small&width=59&height=20&appId" width="59" height="20" style="border:none;overflow:hidden" scrolling="no" frameborder="0" allowTransparency="true" allow="encrypted-media"></iframe>';
$('.facebookshare').append(html);
$('.gallery-lb').each(function() { // the containers for all your galleries
$(this).magnificPopup({
delegate: 'a', // the selector for gallery item
type: 'image',
gallery: {
enabled:true
},
mainClass: 'mfp-fade'
});
});
$('.wrap-slick3').each(function(){
var ele = $(this).find('.slick3');
if (ele !== null){
ele.slick({
slidesToShow: 1,
slidesToScroll: 1,
fade: true,
infinite: true,
autoplay: false,
autoplaySpeed: 6000,
arrows: true,
appendArrows: $(this).find('.wrap-slick3-arrows'),
prevArrow:'<button class="arrow-slick3 prev-slick3"><i class="fa fa-angle-left" aria-hidden="true"></i></button>',
nextArrow:'<button class="arrow-slick3 next-slick3"><i class="fa fa-angle-right" aria-hidden="true"></i></button>',
dots: true,
appendDots: $(this).find('.wrap-slick3-dots'),
dotsClass:'slick3-dots',
customPaging: function(slick, index) {
var portrait = $(slick.$slides[index]).data('thumb');
return '<img src=" ' + portrait + ' "/><div class="slick3-dot-overlay"></div>';
},
});
}
});
}
},
error: function(data){
console.log(data);
}
});
});
$(document).off('click','.js-hide-modal1');
$(document).on('click','.js-hide-modal1',function(){
$('.js-modal1').removeClass('show-modal1');
$('.gallery-lb').slick('unslick');
$('.gallery-lb').empty();
$('.detail-text').empty();
});
//end quick view
//added by vanna
/*===================================================================[ Load more ]*/
$(document).off('click','#loadmore');
$(document).on('click', '#loadmore', function(){
var offset = parseInt($('#offset').val());
var seller_id = $(this).data('seller_id');
var features = $(this).data('features');
if (seller_id === undefined){
seller_id =0;
}
if (features === undefined){
features = 0;
}
//alert(window.location.protocol +'//'+window.location.host+"/admin/product/getproductmore/");
$.ajax({
type:"GET",
url:window.location.protocol +'//'+window.location.host+"/admin/product/getproductmore/",
data:{ offset: offset , seller : seller_id , features:features} ,
success: function (data) {
console.log(data);
if(data[0] == 1){
var i;
var items = data[2];
var $content;
for (i=0; i< items.length; i ++){
$content = $(items[i]);
$('.isotope-grid').append( $content )
.isotope( 'insert', $content );
}
offset = offset +items.length;
$('#offset').val(offset);
}
$('.totalSize').val(data[1]);
updateSize();
},
error: function(data){
console.log(data);
}
});
});
$(document).off('click','#loadmore_shop');
$(document).on('click', '#loadmore_shop', function(){
var offset = parseInt($('#offset').val());
//alert(offset);
$.ajax({
type:"GET",
url:"admin/seller/getsellermore/",
data:{ offset: offset } ,
success: function (data) {
console.log(data);
if(data[0] == 1){
var i;
var items = data[2];
var $content;
for (i=0; i< items.length; i ++){
$content = $(items[i]);
$('.isotope-grid').append( $content )
.isotope( 'insert', $content );
}
offset = offset +items.length;
$('#offset').val(offset);
}
updateSizeShop();
},
error: function(data){
console.log(data);
}
});
});
/*===================================================================[ sort by ]*/
$topeContainer.isotope({
getSortData: {
product_id: '[data-product_id] parseInt',
name: function (itemElem){
var name = $(itemElem).find('.pname').text();
return name.toLowerCase();
},
price:function( itemElem ) { // function
var price = $(itemElem).find('.price').text();
return parseFloat(price);
}
},
});
$(document).off('click','.sort-by');
$(document).on('click','.sort-by', function(){
var sort_by = $(this).data('sort');
var old_sort_by = $('.filter-link-active.sort-by');
old_sort_by.removeClass('filter-link-active');
$(this).addClass('filter-link-active');
if(sort_by == 'default'){
$topeContainer.isotope({
sortBy: ''
});
}else if(sort_by == 'name'){
$topeContainer.isotope({
sortBy: 'name',
sortAscending: true
});
}else if(sort_by == 'newness'){
$topeContainer.isotope({
sortBy: 'product_id',
sortAscending: false
});
}else if (sort_by == 'hightolow'){
$topeContainer.isotope({
sortBy: 'price',
sortAscending: true
});
}else if (sort_by == 'lowtohigh'){
$topeContainer.isotope({
sortBy: 'price',
sortAscending: false
});
}
});
/*===================================================================[ filter by ]*/
$(document).off('click','.filter-by');
$(document).on('click','.filter-by', function(){
var old_active = $('.filter-link-active.filter-by');
$(this).addClass('filter-link-active');
old_active.removeClass('filter-link-active');
$('.filter-price').val($(this).data('filter'));
$topeContainer.isotope({
filter: function() {
return perform_filter($(this));
}
});
updateSize();
})
/*===================================================================[ search by name ]*/
$(document).off('keyup','#search_name');
$(document).on('keyup','#search_name', function(){
$topeContainer.isotope({
filter: function() {
return perform_filter($(this));
}
});
updateSize();
});
function updateSize(){
var totalSize = $('#totalSize').val();
var loaded = $('#offset').val() ;
var displayed = $('.isotope-grid').data('isotope').filteredItems.length;
$('.loaded-report').text( loaded
+ ' out of ' + totalSize + ' products loaded. '
+ ((displayed<loaded)? displayed + ' products displayed after filtered.' : '')
);
}
$(document).off('keyup','#search_shop_name');
$(document).on('keyup','#search_shop_name', function(){
$topeContainer.isotope({
filter: function() {
var filter_by_name = $('#search_shop_name').val().trim().toLowerCase().split(' ');
var shop_name = $(this).find('.sname').text().toLowerCase();
var i;
if (filter_by_name.length == 0)
return true;
else {
for (i =0; i < filter_by_name.length ; i++){
if (shop_name.indexOf(filter_by_name[i] ) != -1){
return true;
}
}
}
return false;
}
});
updateSizeShop();
});
function updateSizeShop(){
var totalSize = $('#totalSize').val();
var loaded = $('#offset').val() ;
var displayed = $('.isotope-grid').data('isotope').filteredItems.length;
$('.loaded-report').text( loaded
+ ' out of ' + totalSize + ' shops loaded. '
+ ((displayed<loaded)? displayed + ' shops displayed after filtered.' : '')
);
}
})(jQuery);
<file_sep>/routes/breadcrumbs/backend/category.php
<?php
Breadcrumbs::for('category.index', function ($trail) {
$trail->push( 'List Category', route('category.index'));
});
Breadcrumbs::for('category.create', function ($trail) {
$trail->parent('category.index');
$trail->push( 'Create Category', route('category.create'));
});
Breadcrumbs::for('category.edit', function ($trail,$id) {
$trail->parent('category.index');
$trail->push( 'Create Category', route('category.edit',$id));
});
Breadcrumbs::for('category.showrate', function ($trail) {
$trail->parent('category.index');
$trail->push( 'Rate Category', route('category.rate'));
});
Breadcrumbs::for('category.rate', function ($trail) {
$trail->parent('category.index');
$trail->parent('category.showrate');
$trail->push( 'Show Rate', route('category.showrate'));
});
| 4bebd0533d6556282eeee583b19938625f1bb37d | [
"JavaScript",
"PHP"
] | 23 | PHP | chhuovanna/secondhand | 3e5d609656aa2c90c7927280bfb4d5914db1c2e9 | b6dc4753c1f15b14de6cf87586e7840e46f34227 | |
refs/heads/master | <repo_name>tkggusraqk/alloy-ui<file_sep>/src/aui-button/tests/unit/js/tests.js
YUI.add('aui-button-tests', function(Y) {
var suite = new Y.Test.Suite('aui-button'),
searchButtonCancel;
function assertPosition(input) {
var inputRegion = input.get('region'),
iconClose = input.previous('.btn-search-cancel'),
xpos = inputRegion.right -
searchButtonCancel.get('iconWidth') +
searchButtonCancel.get('gutter.0'),
ypos = inputRegion.top +
inputRegion.height / 2 -
searchButtonCancel.get('iconHeight') / 2 +
searchButtonCancel.get('gutter.1');
Y.Assert.areSame(
Math.round(iconClose.getY()), Math.round(ypos),
'Y-Position of input and search-btn-cancel should be the same.'
);
Y.Assert.areSame(
iconClose.getX(),
xpos,
'X-Position of input and search-btn-cancel should be the same.'
);
input.simulate('blur');
}
function fillInput(input) {
input.val('This is a test!');
// TODO: Remove this when yeti is fixed to stop stealing focus from the test.
searchButtonCancel._syncButtonUI(input);
}
suite.add(new Y.Test.Case({
name: 'Search Button Cancel',
'#1: Focusing first input should position .btn-search-cancel on the right.': function() {
var test = this,
inputNode = Y.all('input.clearable').item(0);
searchButtonCancel = new Y.ButtonSearchCancel({
trigger: '.clearable'
});
setTimeout(function() {
test.resume(function() {
assertPosition(inputNode);
});
}, 800);
Y.soon(function() {
fillInput(inputNode);
});
test.wait(1000);
},
'#2: Focusing second input should position .btn-search-cancel on the right.': function() {
var test = this,
inputNode = Y.all('input.clearable').item(1);
searchButtonCancel = new Y.ButtonSearchCancel({
trigger: '.clearable'
});
setTimeout(function() {
test.resume(function() {
assertPosition(inputNode);
});
}, 800);
Y.soon(function() {
fillInput(inputNode);
});
test.wait(1000);
},
'#3: .btn-search-cancel should be positioned correctly when input is moved by removing from dom and placing in a different area': function() {
searchButtonCancel = new Y.ButtonSearchCancel({
trigger: '.clearable'
});
Y.one('#search-btn-cancel-heading').insert(
Y.one('#search-btn-cancel-demo'), 'before');
Y.all('input.clearable').each(function(input) {
// TODO: Remove this when yeti is fixed to stop stealing focus from the test.
searchButtonCancel._syncButtonUI(input);
assertPosition(input);
});
}
}));
Y.Test.Runner.add(suite);
}, '', {
requires: ['aui-button', 'aui-button-search-cancel', 'aui-node', 'node-event-simulate', 'test']
});
| 199c13c64d51b4d514ab59a0fba936a5e4db3b27 | [
"JavaScript"
] | 1 | JavaScript | tkggusraqk/alloy-ui | 246700a4af30113ce242afbb8136134554d814a4 | bac329053bef3c877dfc5a1e54e28d2f649e1832 | |
refs/heads/master | <file_sep># -*- coding: utf-8 -*-
"""
Created on Wed Nov 28 21:21:08 2018
@author: melan
"""
from Perform_Analysis import get_commits, read_file
#Testing the code
import unittest
class TestCommits(unittest.TestCase):
def setUp(self):
self.data = read_file('C:\\Users\\melan\\OneDrive\\Documents\\CA4_10390914\\changes_python.log')
def test_number_of_lines(self):
self.assertEqual(5255, len(self.data))
def test_number_of_commits(self):
commits = get_commits(self.data)
commits = get_commits(self.data)
self.assertEqual(422, len(commits))
self.assertEqual('Thomas', commits[0].author)
self.assertEqual('Jimmy', commits[420].author)
if __name__ == '__main__':
unittest.main()<file_sep># -*- coding: utf-8 -*-
"""
Created on Wed Nov 28 22:11:35 2018
@author: melan
"""
class Commit(object):
def __init__(self, revision, author, date, time, number_of_lines, changed_path=[], comment=[]):
self.revision = revision
self.author = author
self.date = date
self.time = time
self.number_of_lines = number_of_lines
self.changed_path = changed_path
self.comment = comment
def __repr__(self):
return self.revision + ',' + self.author + \
',' + self.date + ',' + self.time + ',' + str(self.number_of_lines) + \
',' + ' '.join(self.comment) + '\n'
def get_commits(data):
sep = 72*'-'
commits = []
index = 0
while index < len(data):
try:
# parse each of the commits and put them into a list of commits
details = data[index + 1].split('|')
# the author with spaces at end removed.
commit = Commit(details[0].strip(),
details[1].strip(),
details[2].strip().split(' ')[0],
details[2].strip().split(' ')[1],
int(details[3].strip().split(' ')[0]))
change_file_end_index = data.index('', index + 1)
commit.changed_path = data[index + 3 : change_file_end_index]
commit.comment = data[change_file_end_index + 1 :
change_file_end_index + 1 + commit.number_of_lines]
# add details to the list of commits.
commits.append(commit)
index = data.index(sep, index + 1)
except IndexError:
index = len(data)
return commits
def read_file(any_file):
# use strip to strip out spaces and trim the line.
return [line.strip() for line in open(any_file, 'r')]
def save_commits(commits, any_file):
my_file = open(any_file, 'w')
my_file.write("revision,author,date,time,number_of_lines,comment\n")
for commit in commits:
my_file.write(str(commit))
my_file.close()
if __name__ == '__main__':
# open the file - and read all of the lines.
changes_file = 'C:\\Users\\melan\\OneDrive\\Documents\\CA4_10390914\\changes_python.log'
data = read_file(changes_file)
print(len(data))
commits = get_commits(data)
print(len(commits))
print(commits[0])
print(commits[0].author)
save_commits(commits, 'changes.csv')
#interesting stat no.1
authorNames = set(get_commits("author"))
len(authorNames)
#interesting stat no.2
import pandas as pd
df = pd.DataFrame([get_commits])
df = pd.DataFrame.from_dict(get_commits["author"])
ax = df.plot(kind='bar', title = 'No of comments per author', figsize=(20,10))
ax.set_xlabel('Users',fontsize=14)
ax.set_ylabel('Frequency',fontsize=14)
#interesting stat no.3 | f0d046cf0235ac7a560e9e1ed7e81b033a9b02c6 | [
"Python"
] | 2 | Python | MelanieDBS/CA4_Big_Data | 0829f5a3a4d3e3a67e168e07be128eb2ba8b59ad | c1f1167bed1251c4056355c58be9d0490cfa4401 | |
refs/heads/master | <repo_name>JoekingCooper/project_attenuation<file_sep>/README.md
# project_attenuation
This is an mysterious project with no information ...
<file_sep>/notebooks/.ipynb_checkpoints/breed_generation-checkpoint.py
import numpy as np
import random
import os
import pickle
class BreedGeneration:
"""
A class just used too create the first generation of fighters fr the tournament
"""
def __init__(self,dead_generation,mutation_rate = 0.1,number_of_fighters = 16, last_name_list_file = 'last-names.txt',first_name_list_file = 'first-names.txt'):
"""
Initialize some values mostly with nothings
"""
self.dead_generation = dead_generation
self.generation_number = dead_generation['generation_number']+1
self.cwd = os.getcwd()
self.matrix_dimensions = dead_generation['fighters'][list(dead_generation['fighters'].keys())[1]]['attributes'].shape
self.ids = None
self.generation_dict = dict()
self.number_of_fighters = number_of_fighters
self.file_last_name_list = last_name_list_file
self.file_first_name_list = first_name_list_file
self.mutation_rate = mutation_rate
self.gen_storage_location = os.path.abspath(os.path.join(self.cwd,
'..', 'storage',
'generation_'+str(self.generation_number)+'_pool',
'gen'+str(self.generation_number)+'begin.pickle'))
def createIDList(self):
self.ids = ["g"+str(self.generation_number)+"_c" + str(num) for num in range(self.number_of_fighters)]
def pullNameList(self):
last_name_list_path = os.path.abspath(os.path.join(self.cwd, '..', 'storage','resources',self.file_last_name_list))
text_file = open(last_name_list_path, "r")
self.last_name_list = text_file.read().split('\n')
text_file.close()
first_name_list_path = os.path.abspath(os.path.join(self.cwd, '..', 'storage','resources',self.file_first_name_list))
text_file = open(first_name_list_path, "r")
self.first_name_list = text_file.read().split('\n')
text_file.close()
def populateProbabilities(self):
self.indexlist = list(self.dead_generation['fighters'].keys())
wins = []
for key in self.indexlist:
wins.append(self.dead_generation['fighters'][key]['wins'])
self.fighter_probabilities = np.array(wins)/sum(wins)
def get_matrix_mask_2(self):
mask_test = np.full(self.matrix_dimensions[0]**2,True)
mask_test[0:int(self.matrix_dimensions[0]**2/2)] = False
np.random.shuffle(mask_test)
return mask_test.reshape(self.matrix_dimensions)
def breedFighters(self):
fighter_1 = self.dead_generation['fighters'][np.random.choice(self.indexlist, p=self.fighter_probabilities)]
fighter_2 = self.dead_generation['fighters'][np.random.choice(self.indexlist, p=self.fighter_probabilities)]
#get name
new_first_name = random.choice(self.first_name_list)
new_last_name = random.choice([fighter_1['last_name'],fighter_2['last_name']])
name = new_first_name+' '+new_last_name
#get masks for equation
tf1_mask = self.get_matrix_mask_2()
tf2_mask = np.invert(tf1_mask)
#create base stats
base_fighter_attributes = (fighter_1['attributes']*tf1_mask+fighter_2['attributes']*tf2_mask)
base_fighter_attributes = np.nan_to_num(base_fighter_attributes)
#mutate base stats
mutation = np.random.rand(self.matrix_dimensions[0],self.matrix_dimensions[0])-0.5
new_fighter_attributes = base_fighter_attributes + mutation*self.mutation_rate
return name, new_last_name, new_fighter_attributes
def populateDictionary(self):
self.generation_dict['generation_number']=self.generation_number
self.generation_dict['mutation_rate']=self.mutation_rate
self.generation_dict['fighters']=dict()
for num,i in enumerate(self.ids):
self.generation_dict['fighters'][i] = dict()
#create name
name,last_name, attributes = self.breedFighters()
#other attributes
self.generation_dict['fighters'][i]['name'] = name
self.generation_dict['fighters'][i]['last_name'] = last_name
self.generation_dict['fighters'][i]['alive'] = 1
self.generation_dict['fighters'][i]['attributes'] = attributes
self.generation_dict['fighters'][i]['wins'] = 0
self.generation_dict['fighters'][i]['tournament_count'] = 0
def saveDictionary(self):
with open(self.gen_storage_location, 'wb') as handle:
pickle.dump(self.generation_dict, handle, protocol=pickle.HIGHEST_PROTOCOL)
def pipeline(self):
self.createIDList()
self.pullNameList()
self.populateProbabilities()
self.populateDictionary()
self.saveDictionary()
<file_sep>/notebooks/alpha_motive_tournament.py
import numpy as np
import random
import pickle
def number_of_rounds(n):
return np.floor(np.log(n)/np.log(2))
"""
DONE:
- reset wins and reset life function so that tournament can be rerun
- test with different matrix sizes
TODO:
- combination of attributes as decider (instead of one) + a weight matrix for that combination
"""
class AlphaMotiveTournament():
"""
The tournament class, hosted by AlphaMotive.
"""
def __init__(self,fighter_dict:"Dictionary with fighter data."):
"""
Initialize for the tournament
"""
self.pool_dead = []
self.pool_2 = []
self.fighter_dict = fighter_dict.copy()
self.number_of_rounds = number_of_rounds(len(fighter_dict['fighters']))
self.pool_1 = list(self.fighter_dict['fighters'].keys())
self.pool_init = list(self.fighter_dict['fighters'].keys())
self.fight_number = 0
dummy_attribute_matrix = self.fighter_dict['fighters'][self.pool_1[0]]['attributes']
self.att_matrix_dimension_0 = len(dummy_attribute_matrix)-1
self.att_matrix_dimension_1 = len(dummy_attribute_matrix[0])-1
def single_fight(self,pool,printswitch=True):
contestant1 = random.choice(pool)
pool.remove(contestant1)
contestant2 = random.choice(pool)
pool.remove(contestant2)
#get indx mapping for fight, random values are compared to decide the winner.
inx_0 = random.randint(0, self.att_matrix_dimension_0)
inx_1 = random.randint(0, self.att_matrix_dimension_1)
#print names of contestants
if printswitch:
print('... Fight',self.fight_number)
print('... - ',self.fighter_dict['fighters'][contestant1]['name'],'Vs.',self.fighter_dict['fighters'][contestant2]['name'])
#access the comparison fight values
contestant1_score = self.fighter_dict['fighters'][contestant1]['attributes'][inx_0][inx_1]
contestant2_score = self.fighter_dict['fighters'][contestant2]['attributes'][inx_0][inx_1]
# give winner and looser
winner = np.where(contestant1_score>=contestant2_score,contestant1,contestant2).item()
if printswitch:
print('... - ',self.fighter_dict['fighters'][winner]['name'],'is the winner!')
loser = np.where(contestant1_score<contestant2_score,contestant1,contestant2).item()
return winner, loser
def tournament(self,printswitch=True):
winner = None
pool_1 = self.pool_1
pool_dead = self.pool_dead
pool_2 = self.pool_2
self.fight_number = 0
for tournament_round in range(int(self.number_of_rounds)):
#print stage number
if printswitch:
print('Stage:',tournament_round)
for fight_num in range(int(len(pool_1)/2)):
#run fight
self.fight_number += 1
winner, loser = self.single_fight(pool_1,printswitch=printswitch)
# add winner to next pool
pool_2.append(winner)
self.fighter_dict['fighters'][winner]['wins']+=1
# add loser to pool dead pool
pool_dead.append(loser)
self.fighter_dict['fighters'][loser]['alive']=0
self.fighter_dict['fighters'][loser]['tournament_count']+=1
pool_1 = pool_2.copy()
pool_2 = []
self.pool_1 = pool_1
self.pool_2 = pool_2
self.pool_dead = pool_dead
self.tournament_winner = winner
self.tournament_winner_stats = self.fighter_dict['fighters'][winner]['attributes']
self.fighter_dict['fighters'][winner]['tournament_count']+=1
if printswitch:
print('The Alpha Motive Champion is',self.fighter_dict['fighters'][self.tournament_winner]['name'])
print('Kills =',self.fighter_dict['fighters'][self.tournament_winner]['wins'])
def produceAverageWinner(self):
fights = self.fighter_dict['fighters'][self.tournament_winner]['wins']
attribute_addition = self.fighter_dict['fighters'][self.tournament_winner]['wins']*self.fighter_dict['fighters'][self.tournament_winner]['attributes']
for fighter in self.pool_dead:
attribute_addition += self.fighter_dict['fighters'][fighter]['wins']*self.fighter_dict['fighters'][fighter]['attributes']
fights += self.fighter_dict['fighters'][fighter]['wins']
self.averagewinner_stats = attribute_addition/fights
def resetPools(self):
self.pool_dead = []
self.pool_2 = []
self.pool_1 = list(self.fighter_dict['fighters'].keys())
def resetAlive(self):
for fighter in list(self.fighter_dict['fighters'].keys()):
self.fighter_dict['fighters'][fighter]['alive']=1
def resetWins(self):
for fighter in list(self.fighter_dict['fighters'].keys()):
self.fighter_dict['fighters'][fighter]['wins']=0
def resetAll(self):
self.resetPools()
self.resetAlive()
self.resetWins()
<file_sep>/notebooks/create_gen_0.py
import numpy as np
import random
import os
import pickle
class CreateGeneration0:
"""
A class just used too create the first generation of fighters fr the tournament
"""
def __init__(self,number_of_fighters = 16, last_name_list_file = 'last-names.txt',first_name_list_file = 'first-names.txt',attribute_dimensions = 2):
"""
Initialize some values mostly with nothings
"""
self.cwd = os.getcwd()
self.ids = None
self.name_list = None
self.generation0 = dict()
self.number_of_fighters = number_of_fighters
self.file_last_name_list = last_name_list_file
self.file_first_name_list = first_name_list_file
self.attribute_dimensions = attribute_dimensions
self.gen0_storage_location = os.path.abspath(os.path.join(self.cwd, '..', 'storage','base','data_dict_gen0.pickle'))
def createIDList(self):
self.ids = ["g1_c" + str(num) for num in range(self.number_of_fighters)]
def pullNameList(self):
last_name_list_path = os.path.abspath(os.path.join(self.cwd, '..', 'storage','resources',self.file_last_name_list))
text_file = open(last_name_list_path, "r")
self.last_name_list = text_file.read().split('\n')
text_file.close()
first_name_list_path = os.path.abspath(os.path.join(self.cwd, '..', 'storage','resources',self.file_first_name_list))
text_file = open(first_name_list_path, "r")
self.first_name_list = text_file.read().split('\n')
text_file.close()
def populateDictionary(self):
self.generation0['generation_number']=0
self.generation0['mutation_rate']=0
self.generation0['fighters']=dict()
for num,i in enumerate(self.ids):
self.generation0['fighters'][i] = dict()
#create name
last_name = random.choice(self.last_name_list)
first_name = random.choice(self.first_name_list)
self.generation0['fighters'][i]['name'] = first_name+' '+last_name
self.generation0['fighters'][i]['last_name'] = last_name
#remove used names
self.first_name_list.remove(first_name)
self.last_name_list.remove(last_name)
#other attributes
self.generation0['fighters'][i]['alive'] = 1
self.generation0['fighters'][i]['attributes'] = np.random.rand(self.attribute_dimensions,self.attribute_dimensions)
self.generation0['fighters'][i]['wins'] = 0
self.generation0['fighters'][i]['tournament_count'] = 0
def saveDictionary(self):
with open(self.gen0_storage_location, 'wb') as handle:
pickle.dump(self.generation0, handle, protocol=pickle.HIGHEST_PROTOCOL)
def pipeline(self):
self.createIDList()
self.pullNameList()
self.populateDictionary()
self.saveDictionary() | 6ee13ca1cf56a158b78d9acf5feb305915cdafc5 | [
"Markdown",
"Python"
] | 4 | Markdown | JoekingCooper/project_attenuation | d6ac9c16d4d28e7c63c11241e8e3fa98fe996019 | cbbe964ed4ca978f1ad0e5a21a2f85458435abe5 | |
refs/heads/main | <repo_name>khamulbr/books-ws<file_sep>/src/main/java/com/nazgulsoft/apis/booksws/security/UserDetailsServiceImpl.java
package com.nazgulsoft.apis.booksws.security;
import com.nazgulsoft.apis.booksws.user.User;
import com.nazgulsoft.apis.booksws.user.UserService;
import org.springframework.security.core.userdetails.UserDetails;
import org.springframework.security.core.userdetails.UserDetailsService;
import org.springframework.security.core.userdetails.UsernameNotFoundException;
import org.springframework.stereotype.Service;
@Service
public class UserDetailsServiceImpl implements UserDetailsService {
private UserService userService;
public UserDetailsServiceImpl(UserService userService) {
this.userService = userService;
}
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
User user = userService.getUserByUsername(username);
if (user != null)
return user;
throw new UsernameNotFoundException(username + " does not exist");
}
}
<file_sep>/src/main/resources/application.properties
server.ssl.key-store=serverkeystore.jks
server.ssl.key-store-type=JKS
server.ssl.key-store-password=<PASSWORD>
server.ssl.key-alias=tcserver
<file_sep>/README.md
# books-ws
Code for Spring security code
<file_sep>/src/test/java/com/nazgulsoft/apis/booksws/book/BookControllerIT.java
package com.nazgulsoft.apis.booksws.book;
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.autoconfigure.web.servlet.AutoConfigureMockMvc;
import org.springframework.boot.test.autoconfigure.web.servlet.WebMvcTest;
import org.springframework.security.test.context.support.WithMockUser;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.web.servlet.MockMvc;
import org.springframework.test.web.servlet.MvcResult;
import org.springframework.test.web.servlet.request.MockMvcRequestBuilders;
import org.springframework.test.web.servlet.setup.MockMvcBuilders;
import org.springframework.web.context.WebApplicationContext;
import static org.springframework.security.test.web.servlet.setup.SecurityMockMvcConfigurers.springSecurity;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.status;
@AutoConfigureMockMvc
@ContextConfiguration(classes = BookController.class)
@WebMvcTest
class BookControllerIT {
@Autowired
private WebApplicationContext context;
private MockMvc mockMvc;
@BeforeEach
public void setup() {
mockMvc = MockMvcBuilders
.webAppContextSetup(context)
.apply(springSecurity())
.build();
}
@WithMockUser("user")
@Test
public void testGetBookById() throws Exception {
MvcResult result = mockMvc.perform(MockMvcRequestBuilders.get("/v1/books/1234"))
.andExpect(status().isOk())
.andReturn();
System.out.println(result.getResponse().getContentAsString());
}
}<file_sep>/src/test/java/com/nazgulsoft/apis/booksws/user/UserServiceTest.java
package com.nazgulsoft.apis.booksws.user;
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
class UserServiceTest {
private UserService userService = new UserService();
@Test
public void itShouldReturnTheProperUser__WhenAUserIsPresent() {
//Given
User expectedUser = new User("myusername", "$2a$12$5rYnmiswPytMCmenD7BX6OmeYBe1jYFfKP3B5vG5d80K6gkr5Xr6C", true);
//When
User actualUser = userService.getUserByUsername("myusername");
//Then
assertEquals(expectedUser, actualUser);
}
@Test
public void itShouldReturnNull__WhenAUserIsNotPresent() {
//Given
User expectedUser = new User();
//When
User actualUser = userService.getUserByUsername("myusername2");
//Then
assertNull(actualUser);
}
}<file_sep>/src/main/java/com/nazgulsoft/apis/booksws/user/User.java
package com.nazgulsoft.apis.booksws.user;
import org.springframework.security.core.GrantedAuthority;
import org.springframework.security.core.userdetails.UserDetails;
import java.util.Collection;
import java.util.Objects;
public class User implements UserDetails {
private String username;
private String password;
private Boolean enabled;
public User() {
}
public User(String username, String password, Boolean enabled) {
this.username = username;
this.password = <PASSWORD>;
this.enabled = enabled;
}
public String getUsername() {
return username;
}
@Override
public boolean isAccountNonExpired() {
return enabled;
}
@Override
public boolean isAccountNonLocked() {
return enabled;
}
@Override
public boolean isCredentialsNonExpired() {
return enabled;
}
@Override
public boolean isEnabled() {
return enabled;
}
public void setUsername(String username) {
this.username = username;
}
@Override
public Collection<? extends GrantedAuthority> getAuthorities() {
return null;
}
public String getPassword() {
return <PASSWORD>;
}
public void setPassword(String password) {
this.password = <PASSWORD>;
}
public Boolean getEnabled() {
return enabled;
}
public void setEnabled(Boolean enabled) {
this.enabled = enabled;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
User user = (User) o;
return username.equals(user.username) &&
password.equals(user.password) &&
enabled.equals(user.enabled);
}
@Override
public int hashCode() {
return Objects.hash(username, password, enabled);
}
}
| dc3fb1b6b0d79a127af66b72bc264f51839bc15f | [
"Markdown",
"Java",
"INI"
] | 6 | Java | khamulbr/books-ws | 5edce11edd6d964bddbfab333ac6d145766e0dfd | 36dcffd955b77ae877bf7f49860833e549d2fd36 | |
refs/heads/master | <repo_name>rijugeorge/RestAssured-SOAP<file_sep>/src/main/java/OD_PS_Automation/APIPayloads/package-info.java
/**
*
*/
/**
* @author riju.george
*
*/
package OD_PS_Automation.APIPayloads;<file_sep>/src/main/java/OD_PS_Automation/API/WebApiBase.java
package OD_PS_Automation.API;
import java.net.URI;
public interface WebApiBase {
public String serviceURL ="";
public void formRequestXML();
}
<file_sep>/target/classes/global.properties
// UI Configurations
// WebService Configurations
automation.api.test=TRUe
automation.api.authenticateURL=https://webservices.sandbox.orderdynamics.net
automation.api.authenticateServicePath=/AuthenticationService.svc
automation.api.storeId=AE4A82B9-BCEC-4A3E-AE2F-9FD2B16C12DF
automation.api.accessKey=<KEY><file_sep>/src/main/java/OD_PS_Automation/APIPayloads/ParamResolverTestStepScope.java
package OD_PS_Automation.APIPayloads;
public class ParamResolverTestStepScope implements ParamResolverBase{
public String getResolvedParam(String paramName, String paramResolverType) {
// TODO Auto-generated method stub
return null;
}
@Override
public void setParam(String paramScope, String paramName, String paramResolverType) {
// TODO Auto-generated method stub
}
}
<file_sep>/src/main/java/OD_PS_Automation/APIPayloads/ParamResolverBase.java
package OD_PS_Automation.APIPayloads;
public interface ParamResolverBase {
public String paramName = "";
public String paramScope = "";
public String paramResolverType = "";
public String paramResolvedValue = "";
public String getResolvedParam( String paramName, String paramResolverType);
public void setParam( String paramScope, String paramName, String paramResolverType);
}
<file_sep>/src/main/java/OD_PS_Automation/APIPayloads/ParamResolverGlobalScope.java
package OD_PS_Automation.APIPayloads;
import java.util.Properties;
public class ParamResolverGlobalScope implements ParamResolverBase {
private Properties prop;
private ParamResolverGlobalScope(Properties prop) {
this.prop = prop;
}
public String getResolvedParam(String paramName, String paramResolverType) {
String returnValue = (String) prop.getOrDefault(paramName, "GLOBALNOTSET");
if (returnValue.equals("GLOBALNOTSET")) {
return null;
} else {
return returnValue;
}
}
@Override
public void setParam(String paramScope, String paramName, String paramResolverType) {
// TODO Auto-generated method stub
}
}
<file_sep>/src/main/java/OD_PS_Automation/APIPayloads/ParamResolverFactory.java
package OD_PS_Automation.APIPayloads;
import java.util.Properties;
public class ParamResolverFactory {
public ParamResolverFactory() {
// TODO Auto-generated constructor stub
}
}
<file_sep>/src/main/java/OD_PS_Automation/APIPayloads/BulkParamResolver.java
package OD_PS_Automation.APIPayloads;
import java.util.Properties;
public class BulkParamResolver {
public BulkParamResolver(Properties prop) {
// TODO Auto-generated constructor stub
}
public void performBulkResolution(String type, Properties prop) {
switch (type) {
case "Global" :
for (int i =0 ; i <= prop.size() ; i++) {
}
break;
}
}
}
| 3b9beed65c3de92d0b29bd62f743c1086946c89e | [
"Java",
"INI"
] | 8 | Java | rijugeorge/RestAssured-SOAP | 0febdc1be3ee74595f625004998c40fd7d490e59 | 8dd04265408be447b98317ffdf5ee7fce12d1309 | |
refs/heads/main | <file_sep>import { BrowserModule } from '@angular/platform-browser';
import { RouterModule , Routes} from '@angular/router';
import { AppComponent } from './app.component';
import { BugrsModule } from './bugrs/bugrs.module';
import { HttpClientModule } from '@angular/common/http';
import { BugrsSystemComponent } from './bugrs/bugrs-system/bugrs-system.component';
import { NgModule } from '@angular/core';
import { ReactiveFormsModule } from '@angular/forms';
import { BugrsSubmitFormComponent, } from './bugrs/bugrs-submit-form/bugrs-submit-form.component';
import { CommonModulesModule } from './common-modules/common-modules.module';
const routes: Routes = [
{path : '', component: BugrsSystemComponent},
{path : 'submitbug', component: BugrsSubmitFormComponent },
{path : 'submitbug/:id', component: BugrsSubmitFormComponent },
{path : 'listView', component: BugrsSystemComponent }
];
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
BugrsModule,
CommonModulesModule,
HttpClientModule,
RouterModule.forRoot(routes),
ReactiveFormsModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
<file_sep>import { Injectable } from '@angular/core';
import { HttpClient, HttpParams } from '@angular/common/http';
import { Observable } from 'rxjs';
import { ListStruct } from './list-struct';
import { comment } from './comment';
@Injectable({
providedIn: 'root'
})
export class BugrsRetrievalService {
private endpointURL = 'https://bug-report-system-server.herokuapp.com/bugs';
constructor(private http: HttpClient) { }
getBugsList(): Observable<any> {
const httpParams = new HttpParams() .set('sort', `title,desc`)
.set('size', 'all');
return this.http.get(this.endpointURL, {params: httpParams});
}
getBugsSortedList(sortBy: string, orderBy: string): Observable<any> {
const httpParams = new HttpParams() .set('sort', `${sortBy},${orderBy}`)
.set('size', 'all');
return this.http.get(this.endpointURL, {params: httpParams});
}
getSearchResults(title: string, priority: string, reporter: string, status: string): Observable<any> {
const httpParams = new HttpParams() .set('sort', 'title,desc')
.set('size', 'all')
.set('title', `${title}`)
.set('priority', `${priority}`)
.set('reporter', `${reporter}`)
.set('status', `${status}`);
console.log(this.http.get(this.endpointURL, {params: httpParams}));
return this.http.get(this.endpointURL, {params: httpParams});
}
createBug(bug: ListStruct) {
bug.createdAt = Date.now.toString();
return this.http.post(this.endpointURL, bug);
}
getBug(id: string) {
return this.http.get(`${this.endpointURL}/${id}`);
}
update(bug: any): Observable<any> {
bug.updatedAt = Date.now.toString();
let result: Observable<any>;
result = this.http.put(`${this.endpointURL}/${bug.id}`, bug);
return result;
}
deleteBug(id: string, bug: ListStruct) {
const httpParams = new HttpParams().set('id', id);
httpParams.set('title', bug.title);
httpParams.set('description', bug.description);
httpParams.set('priority', bug.priority);
httpParams.set('reporter', bug.reporter);
const options = { params: httpParams };
console.log(`${this.endpointURL}/${id}`);
return this.http.delete(`${this.endpointURL}/${id}`, options);
}
}
<file_sep>import { TestBed } from '@angular/core/testing';
import { BugrsRetrievalService } from './bugrs-retrieval.service';
describe('BugrsRetrievalService', () => {
beforeEach(() => TestBed.configureTestingModule({}));
it('should be created', () => {
const service: BugrsRetrievalService = TestBed.get(BugrsRetrievalService);
expect(service).toBeTruthy();
});
});
| ca0f86563762f0f42ac681121a51ebea573eaa70 | [
"TypeScript"
] | 3 | TypeScript | StavrosArg/BugReportingSystem | 957ee38c72a93ff901392862cbcbc7112425382f | b82df4e0abee9c6a47bada6576760c9401b0bc45 | |
refs/heads/main | <file_sep>
const Engine = Matter.Engine;
const World = Matter.World;
const Bodies = Matter.Bodies;
const Body = Matter.Body;
var ground,paper,dustI;
var wall1,wall2,floor;
function preload()
{
dustI = loadImage("Untitled.png");
}
function setup() {
createCanvas(800, 700);
myEngine = Engine.create();
myWorld = myEngine.world;
ground = new Ground(900,650,1800);
floor = new Dustbin(620,640,200,20);
wall1 = new Dustbin(510,550,20,200);
wall2 = new Dustbin(730,550,15,200);
paper = new Paper(100,350);
}
function draw() {
background(200);
Engine.update(myEngine);
ground.display();
floor.display();
wall1.display();
wall2.display();
paper.display();
imageMode(CENTER);
image(dustI,615,550,395,300);
}
function keyPressed(){
if(keyCode===UP_ARROW){
Matter.Body.applyForce(paper.body,paper.body.position,{x:0.22,y:-0.22});
}
}
| 5714fee75ece8858dba22cbe6f7f711a85489bce | [
"JavaScript"
] | 1 | JavaScript | Sanskaar717/Project-25 | 06c9d1a7cf3d0f87ca2ccdc076f1f089fb6109d3 | 9f11aec6334b994b9bbe5bce66bbe111bbde056e | |
refs/heads/master | <file_sep># arduino_interactive
Ergebnisse der Creative Media Summer School an der FH St.Pölten im Jahr 2017
<file_sep>import { Component } from '@angular/core';
import { IonicPage, NavController, NavParams } from 'ionic-angular';
import { BluetoothSerial } from '@ionic-native/bluetooth-serial';
import { ToastController } from 'ionic-angular';
/**
* Generated class for the FotomindMiniBtPage page.
*
* See http://ionicframework.com/docs/components/#navigation for more info
* on Ionic pages and navigation.
*/
@IonicPage()
@Component({
selector: 'page-fotomind-mini-bt',
templateUrl: 'fotomind-mini-bt.html',
})
export class FotomindMiniBtPage {
public status: string = "no";
constructor(public navCtrl: NavController, public navParams: NavParams, public bluetoothSerial: BluetoothSerial, public toastCtrl: ToastController) {
}
ionViewDidLoad() {
console.log('ionViewDidLoad FotomindMiniBtPage');
}
ionViewWillEnter() {
console.log('ionViewWillEnter FotomindMiniBtPage');
this.checkBluetooth('modi-manuell');
}
takePicture() {
this.bluetoothSerial.write("trigger-camera\n");
}
checkBluetooth(modus){
this.bluetoothSerial.isEnabled().then(
(data)=> {
console.log("Bluetooth is enabled!");
this.bluetoothSerial.isConnected().then((data) => {
this.bluetoothSerial.write(modus + '\n')
this.subscribeBluetooth().subscribe(
(data)=> {
this.onDataReceived(data);
}
);
});
},
(data)=> {
console.log("Bluetooth is disabled!");
});
}
subscribeBluetooth(){
console.log("Subscribe");
return this.bluetoothSerial.subscribe('\n');
}
onDataReceived(data) {
console.log(data);
if(data.indexOf("Modus") == 0){
console.log(data.substr(data.indexOf("-")+1));
this.status = data.substr(data.indexOf("-")+1);
}
if(data.indexOf("photo-taken") == 0){
let toast = this.toastCtrl.create({
message: 'Photo was taken successfully!',
duration: 3000,
position: 'bottom'
});
toast.present();
}
}
}
<file_sep>import { NgModule } from '@angular/core';
import { IonicPageModule } from 'ionic-angular';
import { ZeitrafferPage } from './zeitraffer';
@NgModule({
declarations: [
ZeitrafferPage,
],
imports: [
IonicPageModule.forChild(ZeitrafferPage),
],
exports: [
ZeitrafferPage
]
})
export class ZeitrafferPageModule {}
<file_sep>import { Component } from '@angular/core';
import { IonicPage, NavController, NavParams } from 'ionic-angular';
import { BluetoothSerial } from '@ionic-native/bluetooth-serial';
//import { FotomindMiniBtPage } from '../fotomind-mini-bt/fotomind-mini-bt';
/**
* Generated class for the BluetoothPage page.
*
* See http://ionicframework.com/docs/components/#navigation for more info
* on Ionic pages and navigation.
*/
@IonicPage()
@Component({
selector: 'page-bluetooth',
templateUrl: 'bluetooth.html',
})
export class BluetoothPage {
public var2: string;
public bluetoothDeviceList = [];
constructor(public navCtrl: NavController, public navParams: NavParams, public bluetoothSerial: BluetoothSerial) {
this.checkBluetooth();
}
ionViewDidLoad() {
console.log('ionViewDidLoad BluetoothPage');
}
itemSelected(item: string) {
this.connectBluetoothDevice(item).subscribe((data)=> {
console.log("connected");
this.bluetoothSerial.write('bt-connected\n')
/*this.subscribeBluetooth().subscribe(
(data)=> {
this.onDataReceived(data);
}
);*/
this.navCtrl.parent.select(1);
//this.navCtrl.push(FotomindMiniBtPage);
},
(data)=> {
console.log("not connected");
});
}
checkBluetooth(){
this.bluetoothSerial.isEnabled().then(
(data)=> {
console.log("Bluetooth is enabled!");
this.getAllBluetoothDevices();
},
(data)=> {
console.log("Bluetooth is diabled!");
this.enableBluetooth();
});
}
enableBluetooth(){
this.bluetoothSerial.enable().then(
(data)=> {
console.log("Bluetooth is enabled!");
this.getAllBluetoothDevices();
},
(data)=> {
console.log("The user did *not* enable Bluetooth");
});
}
isBluetoothConnected(){
this.bluetoothSerial.isConnected().then((data) => {
return true;
},
(data) => {
return false;
});
}
getAllBluetoothDevices(){
this.bluetoothSerial.list().then((allDevices) => {
this.bluetoothDeviceList = allDevices;
if(!(this.bluetoothDeviceList.length > 0)){
this.var2 = "could not find any bluetooth devices";
}
});
}
connectBluetoothDevice(deviceID) {
console.log("Requesting connection to " + deviceID);
return this.bluetoothSerial.connect(deviceID);
}
disconnectBluetooth() {
console.log("trennen");
this.bluetoothSerial.disconnect().then(
(data)=> {
console.log(data);
});
}
subscribeBluetooth(){
//bluetoothSerial.unsubscribe(console.log("Unsuscribe Success"), console.log("Unsuscribe Error"));
console.log("Subscribe");
return this.bluetoothSerial.subscribe('\n');
}
onDataReceived(data) {
console.log(data);
if(data.indexOf("Modus") == 0){
console.log(data.substr(data.indexOf("-")+1));
//$scope.setActualModus(message.substr(message.indexOf("-")+1));
}
}
}
<file_sep>import { NgModule } from '@angular/core';
import { IonicPageModule } from 'ionic-angular';
import { SelbstausloeserPage } from './selbstausloeser';
@NgModule({
declarations: [
SelbstausloeserPage,
],
imports: [
IonicPageModule.forChild(SelbstausloeserPage),
],
exports: [
SelbstausloeserPage
]
})
export class SelbstausloeserPageModule {}
<file_sep>import { Component } from '@angular/core';
import { IonicPage, NavController, NavParams } from 'ionic-angular';
import { BluetoothSerial } from '@ionic-native/bluetooth-serial';
/**
* Generated class for the SelbstausloeserPage page.
*
* See http://ionicframework.com/docs/components/#navigation for more info
* on Ionic pages and navigation.
*/
@IonicPage()
@Component({
selector: 'page-selbstausloeser',
templateUrl: 'selbstausloeser.html',
})
export class SelbstausloeserPage {
status: string = "no";
timerDelay: number = 10;
timerPictures: number = 1;
constructor(public navCtrl: NavController, public navParams: NavParams, public bluetoothSerial: BluetoothSerial) {
}
ionViewDidLoad() {
console.log('ionViewDidLoad SelbstausloeserPage');
}
ionViewWillEnter() {
console.log('ionViewWillEnter SelbstausloeserPage');
this.checkBluetooth('modi-timer');
}
startTimer() {
console.log("timer-start+" + this.timerDelay + "#" + this.timerPictures + "\n");
this.bluetoothSerial.write("timer-start+" + this.timerDelay + "#" + this.timerPictures + "\n");
}
checkBluetooth(modus){
this.bluetoothSerial.isEnabled().then(
(data)=> {
console.log("Bluetooth is enabled!");
this.bluetoothSerial.isConnected().then((data) => {
this.bluetoothSerial.write(modus + '\n')
this.subscribeBluetooth().subscribe(
(data)=> {
this.onDataReceived(data);
}
);
});
},
(data)=> {
console.log("Bluetooth is disabled!");
});
}
subscribeBluetooth(){
console.log("Subscribe");
return this.bluetoothSerial.subscribe('\n');
}
onDataReceived(data) {
console.log(data);
if(data.indexOf("Modus") == 0){
console.log(data.substr(data.indexOf("-")+1));
this.status = data.substr(data.indexOf("-")+1);
}
}
}
<file_sep>import { Component } from '@angular/core';
import { IonicPage, NavController, NavParams } from 'ionic-angular';
import { BluetoothSerial } from '@ionic-native/bluetooth-serial';
/**
* Generated class for the ZeitrafferPage page.
*
* See http://ionicframework.com/docs/components/#navigation for more info
* on Ionic pages and navigation.
*/
@IonicPage()
@Component({
selector: 'page-zeitraffer',
templateUrl: 'zeitraffer.html',
})
export class ZeitrafferPage {
status: string = "no";
timelapseStatus: string = "inaktiv";
timelapseDelayMinutes: number = 0;
timelapseDelaySeconds: number = 10;
timelapseDelay: number = 0;
constructor(public navCtrl: NavController, public navParams: NavParams, public bluetoothSerial: BluetoothSerial) {
}
ionViewDidLoad() {
console.log('ionViewDidLoad ZeitrafferPage');
}
ionViewWillEnter() {
console.log('ionViewWillEnter ZeitrafferPage');
this.checkBluetooth('modi-timelapse');
}
startTimelapse() {
this.timelapseDelay = (this.timelapseDelaySeconds + (this.timelapseDelayMinutes * 60));
console.log(this.timelapseDelay);
this.bluetoothSerial.write("timelapse-start+" + this.timelapseDelay + "\n");
}
stopTimelapse() {
this.bluetoothSerial.write("timelapse-stop\n");
}
checkBluetooth(modus){
this.bluetoothSerial.isEnabled().then(
(data)=> {
console.log("Bluetooth is enabled!");
this.bluetoothSerial.isConnected().then((data) => {
this.bluetoothSerial.write(modus + '\n')
this.subscribeBluetooth().subscribe(
(data)=> {
this.onDataReceived(data);
}
);
});
},
(data)=> {
console.log("Bluetooth is disabled!");
});
}
subscribeBluetooth(){
console.log("Subscribe");
return this.bluetoothSerial.subscribe('\n');
}
onDataReceived(data) {
console.log(data);
if(data.indexOf("Modus") == 0){
console.log(data.substr(data.indexOf("-")+1));
this.status = data.substr(data.indexOf("-")+1);
}
if(data.indexOf("timelapse-active") == 0){
this.setTimelapseStatus("Zeitraffer gestartet!");
console.log(this.timelapseStatus);
}
else if(data.indexOf("timelapse-inactive") == 0){
this.setTimelapseStatus("Zeitraffer gestoppt!");
console.log(this.timelapseStatus);
}
}
setTimelapseStatus(status){
this.timelapseStatus = status;
}
}
<file_sep>#include <Arduino.h>
//INCLUDES
#include <Wire.h>
#include <DS3231.h>
//PINS
const int ledYellowPin = 6;
const int ledGreenPin = 7;
const int potiPin = 2;
const int piezoPin = A0;
const int photoResPin = A1;
const int pirPin = 4;
const int flashPin = 8;
const int shutterPin = 9;
const int focusPin = 10;
const int buttonPin = 11;
const int switchPin = 12;
const int laserPin = 3;
//constant Variables
const int threshold = 500;
//Variables
int potiVal = 0, photoResVal;
int photoResThreshold = 600;
int sensorReading = 0;
int ledState = LOW;
int pirVal;
int shutterDelay = 0;
String message = "";
int buttonState = 0;
int switchState = 0;
int piezoVal = 0;
int lichtschrankeActive = 0;
String actualModi = "Manuell";
DS3231 clock;
RTCDateTime dt;
unsigned long timelapseDelay = 10, timelapseStart, actualTime, timeDiff;
unsigned long timerDelay = 3, timerStart, timerPictureCount = 0, timerPictures= 1;
bool timelapseActive = false, timerActive = false;
String watchControllTime, actualClock;
String test;
// the setup routine runs once when you press reset:
void setup() {
// initialize
pinMode(ledYellowPin, OUTPUT);
pinMode(ledGreenPin, OUTPUT);
pinMode(pirPin, INPUT);
pinMode(potiPin, INPUT);
pinMode(shutterPin, OUTPUT);
pinMode(focusPin, OUTPUT);
pinMode(buttonPin, INPUT);
pinMode(switchPin, INPUT);
pinMode(flashPin, OUTPUT);
pinMode(laserPin, OUTPUT);
Serial.begin(9600);
clock.begin();
clock.setDateTime(__DATE__, __TIME__);
digitalWrite(ledYellowPin, HIGH);
delay(100);
digitalWrite(ledYellowPin, LOW);
digitalWrite(ledGreenPin, HIGH);
delay(100);
digitalWrite(ledGreenPin, LOW);
digitalWrite(ledYellowPin, HIGH);
delay(100);
digitalWrite(ledYellowPin, LOW);
digitalWrite(ledGreenPin, HIGH);
delay(100);
digitalWrite(ledGreenPin, LOW);
analogWrite(laserPin, 150);
delay(200);
analogWrite(laserPin, 0);
actualModi = "Manuell";
//actualModi = "Lichtschranke";
//watchControllTime = "15:02";
//timerStart = seconds();
//timerPictures = 2;
//timerPictureCount = 0;
}
// the loop routine runs over and over again forever:
void loop() {
if(Serial.available()){
message = Serial.readStringUntil('\n');
//Serial.print(message + '\n');
if (message == "trigger-camera")
{
triggerCamera(1000, shutterDelay);
}
else if (message == "modi-manuell")
{
setModi("Manuell");
}
else if (message.indexOf("modi-timelapse") == 0)
{
//Serial.print("Zeitraffer\n");
setModi("Zeitraffer");
}
else if (message.indexOf("timelapse-start") == 0)
{
timelapseDelay = message.substring(message.indexOf('+') + 1).toInt();
timelapseStart = seconds();
timelapseActive = true;
Serial.print("timelapse-started\n");
}
else if (message == "timelapse-stop")
{
timelapseActive = false;
Serial.print("timelapse-stopped\n");
}
else if (message.indexOf("modi-timer") == 0)
{
setModi("Selbstausloeser");
}
else if (message.indexOf("timer-start") == 0)
{
timerDelay = message.substring(message.indexOf('+'), message.indexOf('#')).toInt();
timerPictures = message.substring(message.indexOf('#') + 1).toInt();
timerStart = seconds();
timerPictureCount = 0;
timerActive = true;
}
else if (message == "modi-watchcontroll+20:00")
{
setModi("Uhrzeitsteuerung");
watchControllTime = message.substring(message.indexOf('+') + 1);
}
}
actualTime = seconds();
if(actualModi =="Zeitraffer"){
switchState = digitalRead(switchPin);
if(timelapseActive || switchState == HIGH){
Serial.print("timelapse-active\n");
timeDiff = actualTime - timelapseStart;
if(timeDiff%timelapseDelay == 0){
shootFotoWithoutFocus(500);
delay(600);
}
}
else{
Serial.print("timelapse-inactive\n");
}
}
else if(actualModi =="Uhrzeitsteuerung"){
dt = clock.getDateTime();
actualClock = clock.dateFormat("H:i", dt);
//Serial.println(actualClock);
//Serial.println(watchControllTime);
if(actualClock == watchControllTime){
shootFotoWithoutFocus(200);
}
}
else if(actualModi =="Selbstausloeser" && timerActive){
timeDiff = actualTime - timerStart;
if(timeDiff%timerDelay == 0 && timeDiff > 0 && timerPictureCount < timerPictures){
shootFotoWithoutFocus(200);
delay(600);
timerPictureCount++;
}
if(timerPictureCount == timerPictures){
timerActive = false;
}
}
else if(actualModi == "Lichtschranke"){
buttonState = digitalRead(buttonPin);
switchState = digitalRead(switchPin);
photoResVal = analogRead(photoResPin);
Serial.println(photoResVal);
if (switchState == HIGH)
{
analogWrite(laserPin, 200);
}
else{
analogWrite(laserPin, 0);
}
if (switchState == HIGH && buttonState == HIGH)
{
lichtschrankeActive = 1;
}
if (switchState == HIGH && lichtschrankeActive == 1){
digitalWrite(shutterPin, HIGH);
digitalWrite(ledGreenPin, HIGH);
photoResVal = analogRead(photoResPin);
Serial.print(photoResVal + '\n');
if(photoResVal < photoResThreshold){
delay(25);
shootFlash();
delay(100);
digitalWrite(shutterPin, LOW);
digitalWrite(ledGreenPin, LOW);
lichtschrankeActive = 0;
}
}
else{
digitalWrite(shutterPin, LOW);
digitalWrite(ledGreenPin, LOW);
}
}
else if(actualModi == "Highspeed"){
buttonState = digitalRead(buttonPin);
switchState = digitalRead(switchPin);
photoResVal = analogRead(photoResPin);
Serial.println(photoResVal);
if (switchState == HIGH)
{
analogWrite(laserPin, 200);
}
else{
analogWrite(laserPin, 0);
}
if (switchState == HIGH && buttonState == HIGH)
{
lichtschrankeActive = 1;
}
if (switchState == HIGH && lichtschrankeActive == 1){
if(photoResVal < photoResThreshold){
digitalWrite(shutterPin, HIGH);
digitalWrite(ledGreenPin, HIGH);
delay(10);
digitalWrite(shutterPin, LOW);
digitalWrite(ledGreenPin, LOW);
lichtschrankeActive = 0;
}
}
}
else{
buttonState = digitalRead(buttonPin);
switchState = digitalRead(switchPin);
potiVal = analogRead(potiPin);
shutterDelay = map(potiVal, 0, 1023, 0, 8000);
//Serial.println(shutterDelay);
piezoVal = 1024 - analogRead(piezoPin);
pirVal = digitalRead(pirPin);
//Serial.println(pirVal);
if (buttonState == HIGH && switchState == HIGH)
{
//shootFlash();
triggerCamera(1000, shutterDelay);
}
}
delay(50);
if(actualTime%3 == 0){
Serial.print("Modus-" + actualModi + '\n');
}
}
void setModi(String modus){
actualModi = modus;
Serial.print("Modus-" + actualModi + '\n');
}
unsigned long seconds(){
return millis()/1000;
}
void shootFotoWithoutFocus(int shutterDelayTime){
digitalWrite(shutterPin, HIGH);
digitalWrite(ledGreenPin, HIGH);
delay(shutterDelayTime);
digitalWrite(ledGreenPin, LOW);
digitalWrite(shutterPin, LOW);
delay(100);
}
void shootFlash(){
digitalWrite(flashPin, HIGH);
digitalWrite(ledYellowPin, HIGH);
delay(50);
digitalWrite(ledYellowPin, LOW);
digitalWrite(flashPin, LOW);
delay(50);
}
void triggerCamera(int focusDelay, int shutterDelayTime){
digitalWrite(focusPin, HIGH);
digitalWrite(ledYellowPin, HIGH);
delay(focusDelay);
digitalWrite(focusPin, LOW);
digitalWrite(ledYellowPin, LOW);
delay(200);
digitalWrite(shutterPin, HIGH);
digitalWrite(ledGreenPin, HIGH);
delay(shutterDelayTime);
digitalWrite(ledGreenPin, LOW);
digitalWrite(shutterPin, LOW);
Serial.print("photo-taken\n");
delay(200);
}
<file_sep>import { NgModule, ErrorHandler } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { IonicApp, IonicModule, IonicErrorHandler } from 'ionic-angular';
import { MyApp } from './app.component';
import { BluetoothPage } from '../pages/bluetooth/bluetooth';
import { FotomindMiniBtPage } from '../pages/fotomind-mini-bt/fotomind-mini-bt';
import { ZeitrafferPage } from '../pages/zeitraffer/zeitraffer';
import { SelbstausloeserPage } from '../pages/selbstausloeser/selbstausloeser';
import { TabsPage } from '../pages/tabs/tabs';
import { StatusBar } from '@ionic-native/status-bar';
import { SplashScreen } from '@ionic-native/splash-screen';
import { BluetoothSerial } from '@ionic-native/bluetooth-serial';
import { Toast } from '@ionic-native/toast';
@NgModule({
declarations: [
MyApp,
BluetoothPage,
FotomindMiniBtPage,
ZeitrafferPage,
SelbstausloeserPage,
TabsPage
],
imports: [
BrowserModule,
IonicModule.forRoot(MyApp)
],
bootstrap: [IonicApp],
entryComponents: [
MyApp,
BluetoothPage,
FotomindMiniBtPage,
ZeitrafferPage,
SelbstausloeserPage,
TabsPage
],
providers: [
StatusBar,
SplashScreen,
BluetoothSerial,
Toast,
{provide: ErrorHandler, useClass: IonicErrorHandler}
]
})
export class AppModule {}
<file_sep>import { Component } from '@angular/core';
import { BluetoothPage } from '../bluetooth/bluetooth';
import { FotomindMiniBtPage } from '../fotomind-mini-bt/fotomind-mini-bt';
import { ZeitrafferPage } from '../zeitraffer/zeitraffer';
import { SelbstausloeserPage } from '../selbstausloeser/selbstausloeser';
@Component({
templateUrl: 'tabs.html'
})
export class TabsPage {
tab1Root = BluetoothPage;
tab2Root = FotomindMiniBtPage;
tab3Root = ZeitrafferPage;
tab4Root = SelbstausloeserPage;
constructor() {
}
}
| 4bb3a2e48e407fa8a13b66aee975db10d508aee1 | [
"Markdown",
"TypeScript",
"C++"
] | 10 | Markdown | Woid4tler/arduino_interactive | f87b5f4d460367e7a1d649a81055e7f2a91a80e2 | f590bb5e6ea7017dece0230d581e821e7b5a92e1 | |
refs/heads/master | <file_sep>; Configuration file for the SciTE4AutoHotkey toolbar
; March 1, 2009 - fincs
; Aspect settings for the toolbar
[TOOLBAR]
; Number of rows
rows=16
; Number of colums
cols=4
; Space between icons in pixels
spaces=4
; Icon size in pixels
iconsize=16
; Tool definitions
; They are in the following format:
; =Tool Name|Command line|Hotkey (optional but the vertical bar must be present)|Icon (optional as Hotkey)
; In the command line:
; %FILENAME% represents the filename, no dir
; %FILEPATH% represents the path of the filename
; %FULLFILENAME% represents the full filename of the file (path included)
; %SCITEDIR% represents the directory where SciTE resides
=Active Window Info (Ctrl+1)|..\AU3_Spy.exe|^1|
=Auto Script Writer|..\AutoScriptWriter\AutoScriptWriter.exe||
=autohotkey.net Tool (Ctrl+2)|ahknetutil.exe|^2|
=SmartGUI Creator (Ctrl+3)|tools\SmartGUI.exe|^3|
=MsgBox Creator (Ctrl+4)|tools\MsgBoxC.exe|^4|
=SplashImage Maker (Ctrl+5)|tools\SplashImageM.exe|^5|
=GenDocs Documentation Generator (Ctrl+6)|GenDocs\GenDocs.exe|^6|
=Scriptlet Utility (Ctrl+7)|sutility\sutility.exe|^7|
=Scriptlet: (Example) Run or activate Notepad|"%SCITEDIR%\sutility\sutility.exe" /insert "(Example) Run or activate Notepad"||%SCITEDIR%\sutility\sutility.exe,2<file_sep>[Settings]
ShiftMove=Yes
[Folders]
LoadDir=C:\Users\Scott\Shared Stuff\AutoHotKey\Start Up\Work\Order Helper\GUI
<file_sep>SciTE4AutoHotkey v2.0
=====================
Copy the contents of this archive to:
%AhkPath%\SciTE
where %AhkPath% is the directory where AutoHotkey resides.
If you are using Windows 95/98/ME, delete the following files:
ahk.properties
toolbar.properties
and rename the following files to:
ahk.win9x.properties -> ahk.properties
toolbar.win9x.properties -> toolbar.properties
If you want the PSPad syntax highlighting style,
delete the ahk.style.properties file and rename the
ahk.style.pspad.properties to ahk.style.properties.
If you want to turn off the auto-backup function,
change the following line in ahk.properties:
make.backup=1
to:
make.backup=0
Enjoy!<file_sep>[Options]
; TitleMatchMode=2
[Exclude Windows]
; Window=Virtual PC
; Window=Remote Desktop
[Gather: Exclude Windows]
Window=ahk_class SideBar_AppBarWindow
Window=ahk_class SideBar_HTMLHostWindow
Window=ahk_class BasicWindow
Window=ahk_class _GD_Sidebar
Window=ahk_class PluginHost
Window=ahk_class ATL:051D1598
[Gather: Exclude Processes]
; Process=sidebar.exe
[Hotkeys]
;CapsLock = Hotkeys, Active Window (WADS)
Numpad0 = Hotkeys, Active Window (Numpad)
;NumpadDot = Hotkeys, Previous Window (Numpad)
;XButton2 = Hotkeys, Switch Screen (XButton2), D0.1
XButton2 = Hotkeys, Switch Screen (XButton2)
[Hotkeys: Switch Screen (XButton2)]
XButton2 Up = WindowScreenMove, Next
;WheelUp = WindowScreenMove, Prev
;WheelDown = WindowScreenMove, Next
[Hotkeys: Active Window (WADS)]
z = WindowPadMove, -1, +1, 0.5, 0.5
x = WindowPadMove, 0, +1, 1.0, 0.5
c = WindowPadMove, +1, +1, 0.5, 0.5
a = WindowPadMove, -1, 0, 0.5, 1.0
s = WindowPadMove, +0.25, +0.25, 0.5, 0.5
d = WindowPadMove, +1, 0, 0.5, 1.0
q = WindowPadMove, -1, -1, 0.5, 0.5
w = WindowPadMove, 0, -1, 1.0, 0.5
e = WindowPadMove, +1, -1, 0.5, 0.5
Tab = MaximizeToggle
Space = WindowScreenMove, Next
LAlt = WindowScreenMove, Prev
1 = GatherWindows, 1
2 = GatherWindows, 2
[Hotkeys: Active Window (Numpad)]
*Numpad1 = WindowPadMove, -1, +1, 0.5, 0.5
*Numpad2 = WindowPadMove, 0, +1, 1.0, 0.5
*Numpad3 = WindowPadMove, +1, +1, 0.5, 0.5
*Numpad4 = WindowPadMove, -1, 0, 0.5, 1.0
*Numpad5 = WindowPadMove, +0.25, +0.25, 0.5, 0.5
*Numpad6 = WindowPadMove, +1, 0, 0.5, 1.0
*Numpad7 = WindowPadMove, -1, -1, 0.5, 0.5
*Numpad8 = WindowPadMove, 0, -1, 1.0, 0.5
*Numpad9 = WindowPadMove, +1, -1, 0.5, 0.5
*NumpadAdd = MaximizeToggle
*NumpadEnter = WindowScreenMove, Next
*NumpadDiv = GatherWindows, 1
*NumpadMult = GatherWindows, 2
[Hotkeys: Previous Window (Numpad)]
*Numpad1 = WindowPadMove, -1, +1, 0.5, 0.5, P
*Numpad2 = WindowPadMove, 0, +1, 1.0, 0.5, P
*Numpad3 = WindowPadMove, +1, +1, 0.5, 0.5, P
*Numpad4 = WindowPadMove, -1, 0, 0.5, 1.0, P
*Numpad5 = WindowPadMove, +0.25, +0.25, 0.5, 0.5, P
*Numpad6 = WindowPadMove, +1, 0, 0.5, 1.0, P
*Numpad7 = WindowPadMove, -1, -1, 0.5, 0.5, P
*Numpad8 = WindowPadMove, 0, -1, 1.0, 0.5, P
*Numpad9 = WindowPadMove, +1, -1, 0.5, 0.5, P
*NumpadAdd = MaximizeToggle, P
*NumpadEnter = WindowScreenMove, Next, P
*NumpadDiv = GatherWindows, 1
*NumpadMult = GatherWindows, 2
<file_sep>[Settings]
TimeStart=100
SensitivityAngle=40
SensitivityPoints=5
[Gesture 1]
Name=Copy
SymbolNumber=1
Shortcut=
1=Send, ^c
[Gesture 2]
Name=Paste
SymbolNumber=2
Shortcut=
1=Send, ^v
[Gesture 3]
Name=Cut
SymbolNumber=51
Shortcut=
1=Send, ^x
[Gesture 4]
Name=ShowDesktop
SymbolNumber=125
Shortcut=
1=WinMinimizeAll
[Gesture 5]
Name=Delete
SymbolNumber=111
Shortcut=
1=Send, {Delete}
[Gesture 6]
Name=Undo
SymbolNumber=105
Shortcut=
1=Send, ^z
[Gesture 7]
Name=Redo
SymbolNumber=106
Shortcut=
1=Send, ^y
[Gesture 8]
Name=Minimize
SymbolNumber=8
Shortcut=
1=WinGetTitle, WinTitle, A
2=WinMinimize, %WinTitle%
[Gesture 9]
Name=Maximize
SymbolNumber=5
Shortcut=
1=WinGetTitle, WinTitle, A
2=WinMaximize, %WinTitle%
/* Arrows */
[Symbol 1]
Name=N Arrow
;E
;|
;|
;|
;S
;POS=X.Y
Pos1=0.0
Pos2=0.1
[Symbol 2]
Name=S Arrow
;S
;|
;|
;|
;E
;POS=X.Y
Pos1=0.1
Pos2=0.0
[Symbol 3]
Name=E Arrow
;S---E
;POS=X.Y
Pos1=0.0
Pos2=1.0
[Symbol 4]
Name=W Arrow
;E---S
;POS=X.Y
Pos1=1.0
Pos2=0.0
[Symbol 5]
Name=NE Arrow
; E
; /
; /
; /
;S
;POS=X.Y
Pos1=0.0
Pos2=1.1
[Symbol 6]
Name=NW Arrow
;E
; \
; \
; \
; S
;POS=X.Y
;-------
Pos1=1.0
Pos2=0.1
[Symbol 7]
Name=SE Arrow
;S
; \
; \
; \
; E
;POS=X.Y
Pos1=0.1
Pos2=1.0
[Symbol 8]
Name=SW Arrow
; S
; /
; /
; /
;E
;POS=X.Y
;-------
Pos1=1.1
Pos2=0.0
[Symbol 9]
Name=N-E Arrow
;·---E
;|
;|
;|
;S
;POS=X.Y
Pos1=0.0
Pos2=0.1
Pos3=1.1
[Symbol 10]
Name=N-W Arrow
;E---·
; |
; |
; |
; S
;POS=X.Y
Pos1=1.0
Pos2=1.1
Pos3=0.1
[Symbol 11]
Name=S-E Arrow
;S
;|
;|
;|
;·---E
;POS=X.Y
Pos1=0.1
Pos2=0.0
Pos3=1.0
[Symbol 12]
Name=S-W Arrow
; S
; |
; |
; |
;E---·
;POS=X.Y
Pos1=1.1
Pos2=1.0
Pos3=0.0
[Symbol 13]
Name=E-N Arrow
; E
; |
; |
; |
;S---·
;POS=X.Y
Pos1=0.0
Pos2=1.0
Pos3=1.1
[Symbol 14]
Name=E-S Arrow
;S---·
; |
; |
; |
; E
;POS=X.Y
Pos1=0.1
Pos2=1.1
Pos3=1.0
[Symbol 15]
Name=W-N Arrow
;E
;|
;|
;|
;·---S
;POS=X.Y
Pos1=1.0
Pos2=0.0
Pos3=0.1
[Symbol 16]
Name=W-S Arrow
;·---S
;|
;|
;|
;E
;POS=X.Y
Pos1=1.1
Pos2=0.1
Pos3=0.0
/* Digits */
[Symbol 17]
Name=0
;·---·
;| |
;| |
;E |
;S---·
;POS=X.Y
;-------
Pos1=0.0
Pos2=4.0
Pos3=4.4
Pos4=0.4
Pos5=0.0
[Symbol 18]
Name=0 (Inv)
;·---·
;| |
;| |
;S |
;E---·
;POS=X.Y
;-------
Pos1=0.0
Pos2=0.4
Pos3=4.4
Pos4=4.0
Pos5=0.0
[Symbol 19]
Name=1
; ·
; /|
; S |
; |
; E
;POS=X.Y
;-------
Pos1=0.3
Pos2=2.4
Pos3=2.0
[Symbol 20]
Name=1 (Inv)
; ·
; /|
; E |
; |
; S
;POS=X.Y
;-------
Pos1=2.0
Pos2=2.4
Pos3=0.3
[Symbol 21]
Name=2
;S---·
; |
; ·-·
; /
;·---E
;POS=X.Y
;-------
Pos1=0.4
Pos2=4.4
Pos3=4.2
Pos4=2.2
Pos5=0.0
Pos6=4.0
[Symbol 22]
Name=2 (Inv)
;E---·
; |
; ·-·
; /
;·---S
;POS=X.Y
;-------
Pos1=4.0
Pos2=0.0
Pos3=2.2
Pos4=4.2
Pos5=4.4
Pos6=0.4
[Symbol 23]
Name=2B
;S---·
; |
; ·
;·· /
;··X-E
;POS=X.Y
;-------
Pos1=0.4
Pos2=4.4
Pos3=4.2
Pos4=2.0
Pos5=0.0
Pos6=0.1
Pos7=1.1
Pos8=2.0
Pos9=4.0
[Symbol 24]
Name=2B
;E---·
; |
; ·
;·· /
;··X-S
;POS=X.Y
;-------
Pos1=4.0
Pos2=2.0
Pos3=1.1
Pos4=0.1
Pos5=0.0
Pos6=2.0
Pos7=4.2
Pos8=4.4
Pos9=0.4
[Symbol 25]
Name=3
;S---
; )
; --
; )
;E---
;POS=X.Y
;-------
Pos1=0.4
Pos2=3.4
Pos3=4.3
Pos4=3.2
Pos5=1.2
Pos6=3.2
Pos7=4.1
Pos8=3.0
Pos9=0.0
[Symbol 26]
Name=3 (Inv)
;E---
; )
; --
; )
;S---
;POS=X.Y
;-------
Pos1=0.0
Pos2=3.0
Pos3=4.1
Pos4=3.2
Pos5=1.2
Pos6=3.2
Pos7=4.3
Pos8=3.4
Pos9=0.4
[Symbol 27]
Name=4
; ·
; /|
;·-|-E
; |
; S
;POS=X.Y
;-------
Pos1=2.0
Pos2=2.4
Pos3=0.2
Pos4=4.2
[Symbol 28]
Name=4 (Inv)
; ·
; /|
;·-|-S
; |
; E
;POS=X.Y
;-------
Pos1=4.2
Pos2=0.2
Pos3=2.4
Pos4=2.0
[Symbol 29]
Name=4B
;S ··
;| ||
;·--·|
; |
; E
;POS=X.Y
;-------
Pos1=0.4
Pos2=0.2
Pos3=4.2
Pos4=4.4
Pos5=4.0
[Symbol 30]
Name=4B (Inv)
;E ··
;| ||
;·--·|
; |
; S
;POS=X.Y
;-------
Pos1=4.0
Pos2=4.4
Pos3=4.2
Pos4=0.2
Pos5=0.4
[Symbol 31]
Name=5
;·---S
;|
;·---
; )
;E---
;POS=X.Y
;-------
Pos1=4.4
Pos2=0.4
Pos3=0.2
Pos4=3.2
Pos5=4.1
Pos6=3.0
Pos7=0.0
[Symbol 32]
Name=5 (Inv)
;·---E
;|
;·---
; )
;S---
;POS=X.Y
;-------
Pos1=0.0
Pos2=3.0
Pos3=4.1
Pos4=3.2
Pos5=0.2
Pos6=0.4
Pos7=4.4
[Symbol 33]
Name=6
;·---S
;|
;|E--·
;| |
;·---·
;POS=X.Y
;-------
Pos1=4.4
Pos2=0.4
Pos3=0.0
Pos4=4.0
Pos5=4.2
Pos6=0.2
[Symbol 34]
Name=6 (Inv)
;·---E
;|
;|S--·
;| |
;·---·
;POS=X.Y
;-------
Pos1=0.2
Pos2=4.2
Pos3=4.0
Pos4=0.0
Pos5=0.4
Pos6=4.4
[Symbol 35]
Name=7
;S---·
; /
; /
; /
;E
;POS=X.Y
;-------
Pos1=0.4
Pos2=4.4
Pos3=0.0
[Symbol 36]
Name=7 (Inv)
;S---·
; /
; /
; /
;E
;POS=X.Y
;-------
Pos3=0.0
Pos2=4.4
Pos1=0.4
[Symbol 37]
Name=7B
;S---·
; /
;·--E
;|/
;·
;POS=X.Y
;-------
Pos1=0.4
Pos2=4.4
Pos3=0.0
Pos4=0.2
Pos5=3.2
[Symbol 38]
Name=7B (Inv)
;E---·
; /
;·--S
;|/
;·
;POS=X.Y
;-------
Pos1=3.2
Pos2=0.2
Pos3=0.0
Pos4=4.4
Pos5=0.4
[Symbol 39]
Name=8
;·-S E
;| |
;·---·
;| |
;·---·
;POS=X.Y
;-------
Pos1=2.4
Pos2=0.4
Pos3=0.2
Pos4=4.2
Pos5=4.0
Pos6=0.0
Pos7=0.2
Pos8=4.2
Pos9=4.4
[Symbol 40]
Name=8 (Inv)
;·-E S
;| |
;·---·
;| |
;·---·
;POS=X.Y
;-------
Pos1=4.4
Pos2=4.2
Pos3=0.2
Pos4=0.0
Pos5=4.0
Pos6=4.2
Pos7=0.2
Pos8=0.4
Pos9=2.4
[Symbol 41]
Name=9
;·---S
;| ,
;·---·
; |
;E---·
;POS=X.Y
;-------
Pos1=4.4
Pos2=0.4
Pos3=0.2
Pos4=4.2
Pos5=4.3
Pos6=4.0
Pos7=0.0
[Symbol 42]
Name=9 (Inv)
;·---E
;| ,
;·---·
; |
;S---·
;POS=X.Y
;-------
Pos1=0.0
Pos2=4.0
Pos3=4.3
Pos4=4.2
Pos5=0.2
Pos6=0.4
Pos7=4.4
/* Letters */
[Symbol 43]
Name=A
; ·
; / \
; / \
; /E-- \
;S \--·
;POS=X.Y
;-------
Pos1=0.0
Pos2=2.4
Pos3=4.0
Pos4=1.2
[Symbol 44]
Name=A (Inv)
; ·
; / \
; / \
; /S-- \
;E \--·
;POS=X.Y
;-------
Pos1=1.2
Pos2=4.0
Pos3=2.4
Pos4=0.0
[Symbol 45]
Name=A2
; ·
; / \
; / \
; /E---·\
;S \·
;POS=X.Y
;-------
Pos1=0.0
Pos2=2.4
Pos3=4.0
Pos4=3.2
Pos5=1.2
[Symbol 46]
Name=A2 (Inv)
; ·
; / \
; / \
; /S---·\
;E \·
;POS=X.Y
;-------
Pos1=1.2
Pos2=3.2
Pos3=4.0
Pos4=2.4
Pos5=0.0
[Symbol 47]
Name=A3
; ·
; / \
; / \
; / \
;S E
;POS=X.Y
;-------
Pos1=0.0
Pos2=2.4
Pos3=4.0
[Symbol 48]
Name=A3 (Inv)
; ·
; / \
; / \
; / \
;E S
;POS=X.Y
;-------
Pos1=4.0
Pos2=2.4
Pos3=0.0
[Symbol 49]
Name=B
;S·--
;|| )
;|| |
;|| )
;··E-
;POS=X.Y
;-------
Pos1=0.4
Pos2=0.0
Pos3=0.4
Pos4=2.4
Pos5=3.3
Pos6=2.2
Pos7=3.1
Pos8=2.0
Pos9=0.0
[Symbol 50]
Name=B (Inv)
;E·--
;|| )
;|| |
;|| )
;··S-
;POS=X.Y
;-------
Pos1=0.0
Pos2=2.0
Pos3=3.1
Pos4=2.2
Pos5=3.3
Pos6=2.4
Pos7=0.4
Pos8=0.0
Pos9=0.4
[Symbol 51]
Name=C
;·----S
;|
;|
;|
;·----E
;POS=X.Y
;-------
Pos1=4.4
Pos2=0.4
Pos3=0.0
Pos4=4.0
[Symbol 52]
Name=C (Inv)
;·----E
;|
;|
;|
;·----S
;POS=X.Y
;-------
Pos1=4.0
Pos2=0.0
Pos3=0.4
Pos4=4.4
[Symbol 53]
Name=D
;S·--
;|| \
;|| ·
;|| /
;·· E
;POS=X.Y
;-------
Pos1=0.4
Pos2=0.0
Pos3=0.4
Pos4=3.4
Pos5=4.2
Pos6=3.0
Pos7=0.0
[Symbol 54]
Name=D (Inv)
;E·--
;|| \
;|| ·
;|| /
;·· S
;POS=X.Y
;-------
Pos1=0.0
Pos2=3.0
Pos3=4.2
Pos4=3.4
Pos5=0.4
Pos6=0.0
Pos7=0.4
[Symbol 55]
Name=D2
;·· E
;|| \
;|| ·
;|| /
;S·--
;POS=X.Y
;-------
Pos1=0.0
Pos2=0.4
Pos3=0.0
Pos4=3.0
Pos5=4.2
Pos6=3.4
Pos7=0.4
[Symbol 56]
Name=D2 (Inv)
;·· S
;|| \
;|| ·
;|| /
;E·--
;POS=X.Y
;-------
Pos7=0.4
Pos6=3.4
Pos5=4.2
Pos4=3.0
Pos3=0.0
Pos2=0.4
Pos1=0.0
[Symbol 57]
Name=D3
; E-
;S \
;| ·
;| /
;·--
;POS=X.Y
;-------
Pos1=0.4
Pos2=0.0
Pos3=3.0
Pos4=4.2
Pos5=3.4
Pos6=0.4
[Symbol 58]
Name=D3 (Inv)
; S-
;E \
;| ·
;| /
;·--
;POS=X.Y
;-------
Pos1=0.4
Pos2=3.4
Pos3=4.2
Pos4=3.0
Pos5=0.0
Pos6=0.4
[Symbol 59]
Name=E
;·---S
;|
;·-·
;·-·
;|
;·---E
;POS=X.Y
;-------
Pos1=4.4
Pos2=0.4
Pos3=0.2
Pos4=2.2
Pos5=0.2
Pos6=0.0
Pos7=4.0
[Symbol 60]
Name=E (Inv)
;·---E
;|
;·-·
;·-·
;|
;·---S
;POS=X.Y
;-------
Pos1=4.0
Pos2=0.0
Pos3=0.2
Pos4=2.2
Pos5=0.2
Pos6=0.4
Pos7=4.4
[Symbol 61]
Name=F
;S·--·
;||·-·
;|||
;||·-E
;||
;··
;POS=X.Y
;-------
Pos1=0.4
Pos2=0.0
Pos3=0.4
Pos4=4.4
Pos5=0.4
Pos6=0.2
Pos7=2.2
[Symbol 62]
Name=F (Inv)
;E·--·
;||·-·
;|||
;||·-S
;||
;··
;POS=X.Y
;-------
Pos1=2.2
Pos2=0.2
Pos3=0.4
Pos4=4.4
Pos5=0.4
Pos6=0.0
Pos7=0.4
[Symbol 63]
Name=G
;·---S
;|
;| ·--·
;| ·-·|
;| ||
;·---·E
;POS=X.Y
;-------
Pos1=4.4
Pos2=0.4
Pos3=0.0
Pos4=4.0
Pos5=4.2
Pos6=2.2
Pos7=4.2
Pos8=4.0
[Symbol 64]
Name=G (Inv)
;·---E
;|
;| ·--·
;| ·-·|
;| ||
;·---·S
;POS=X.Y
;-------
Pos1=4.0
Pos2=4.2
Pos3=2.2
Pos4=4.2
Pos5=4.0
Pos6=0.0
Pos7=0.4
Pos8=4.4
[Symbol 65]
Name=H
;S ··
;| ||
;|·---·|
;|| |
;·· E
;POS=X.Y
;-------
Pos1=0.4
Pos2=0.0
Pos3=0.2
Pos4=4.2
Pos5=4.4
Pos6=4.0
[Symbol 66]
Name=H (Inv)
;E ··
;| ||
;|·---·|
;|| |
;·· S
;POS=X.Y
;-------
Pos1=4.0
Pos2=4.4
Pos3=4.2
Pos4=0.2
Pos5=0.0
Pos6=0.4
[Symbol 67]
Name=I
;S
;|
;|
;|
;E
;POS=X.Y
;-------
Pos1=0.4
Pos2=0.0
[Symbol 68]
Name=I (Inv)
;S
;|
;|
;|
;E
;POS=X.Y
;-------
Pos1=0.0
Pos2=0.4
[Symbol 69]
Name=J
; S
; |
; |
; |
;E---·
;POS=X.Y
;-------
Pos1=4.4
Pos2=4.0
Pos3=0.0
[Symbol 70]
Name=J (Inv)
; E
; |
; |
; |
;S---·
;POS=X.Y
;-------
Pos1=0.0
Pos2=4.0
Pos3=4.4
[Symbol 71]
Name=J2
; S-·
; |
; |
; |
;E---·
;POS=X.Y
;-------
Pos1=2.4
Pos1=4.4
Pos2=4.0
Pos3=0.0
[Symbol 72]
Name=J (Inv)
; E-·
; |
; |
; |
;S---·
;POS=X.Y
;-------
Pos1=0.0
Pos2=4.0
Pos3=4.4
Pos4=2.4
[Symbol 73]
Name=K
;S ··
;| //
;| //
;|| \
;|| \
;·· E
;POS=X.Y
;-------
Pos1=0.4
Pos2=0.0
Pos3=0.2
Pos4=4.4
Pos5=0.2
Pos6=4.0
[Symbol 74]
Name=K (Inv)
;E ··
;| //
;| //
;|| \
;|| \
;·· S
;POS=X.Y
;-------
Pos1=4.0
Pos2=0.2
Pos3=4.4
Pos4=0.2
Pos5=0.0
Pos6=0.4
[Symbol 75]
Name=L
;S
;|
;|
;|
;·---E
;POS=X.Y
;-------
Pos1=0.4
Pos2=0.0
Pos3=4.0
[Symbol 76]
Name=L (Inv)
;E
;|
;|
;|
;·---S
;POS=X.Y
;-------
Pos1=4.0
Pos2=0.0
Pos3=0.4
[Symbol 77]
Name=M
;· ·
;|\ /|
;| · |
;| |
;S E
;POS=X.Y
;-------
Pos1=0.0
Pos2=0.4
Pos3=2.2
Pos4=4.4
Pos5=4.0
[Symbol 78]
Name=M (Inv)
;· ·
;|\ /|
;| · |
;| |
;E S
;POS=X.Y
;-------
Pos1=4.0
Pos2=4.4
Pos3=2.2
Pos4=0.4
Pos5=0.0
[Symbol 79]
Name=N
;· E
;|\ |
;| \ |
;| \|
;S ·
;POS=X.Y
;-------
Pos1=0.0
Pos2=0.4
Pos3=4.0
Pos4=4.4
[Symbol 80]
Name=N (Inv)
;· S
;|\ |
;| \ |
;| \|
;E ·
;POS=X.Y
;-------
Pos1=4.4
Pos2=4.0
Pos3=0.4
Pos4=0.0
[Symbol 81]
Name=O
;·-SE-·
;| |
;| |
;| |
;·----·
;POS=X.Y
;-------
Pos1=2.4
Pos2=0.4
Pos3=0.0
Pos4=4.0
Pos5=4.4
Pos6=2.4
[Symbol 82]
Name=O (Inv)
;·-ES-·
;| |
;| |
;| |
;·----·
;POS=X.Y
;-------
Pos1=2.4
Pos2=4.4
Pos3=4.0
Pos4=0.0
Pos5=0.4
Pos6=2.4
[Symbol 83]
Name=P
;S·---·
;|| |
;||E--·
;||
;··
;POS=X.Y
;-------
Pos1=0.4
Pos2=0.0
Pos3=0.4
Pos4=4.4
Pos5=4.2
Pos6=0.2
[Symbol 84]
Name=P (Inv)
;E·---·
;|| |
;||S--·
;||
;··
;POS=X.Y
;-------
Pos1=0.2
Pos2=4.2
Pos3=4.4
Pos4=0.4
Pos5=0.0
Pos6=0.4
[Symbol 85]
Name=P2
;·---·
;| |
;|E--·
;|
;S
;POS=X.Y
;-------
Pos1=0.0
Pos2=0.4
Pos3=4.4
Pos4=4.2
Pos5=0.2
[Symbol 86]
Name=P2 (Inv)
;·---·
;| |
;|S--·
;|
;E
;POS=X.Y
;-------
Pos1=0.2
Pos2=4.2
Pos3=4.4
Pos4=0.4
Pos5=0.0
[Symbol 87]
Name=Q
;·-S·-·
;| | |
;| | |
;| \|
;·----·
; E
;POS=X.Y
;-------
Pos1=2.4
Pos2=0.4
Pos3=0.0
Pos4=4.0
Pos5=4.4
Pos6=2.4
Pos7=2.2
Pos8=4.0
[Symbol 88]
Name=Q (Inv)
;·-E·-·
;| | |
;| | |
;| \|
;·----·
; S
;POS=X.Y
;-------
Pos1=4.0
Pos2=2.2
Pos3=2.4
Pos4=4.4
Pos5=4.0
Pos6=0.0
Pos7=0.4
Pos8=2.4
[Symbol 89]
Name=Q2
;S·---·
;| \ |
;| \ |
;| \|
;·----·
; E
;POS=X.Y
;-------
Pos1=0.4
Pos2=0.0
Pos3=4.0
Pos4=4.4
Pos5=0.4
Pos6=4.0
[Symbol 90]
Name=Q2 (Inv)
;E·---·
;| \ |
;| \ |
;| \|
;·----·
; S
;POS=X.Y
;-------
Pos6=4.0
Pos5=0.4
Pos4=4.4
Pos3=4.0
Pos2=0.0
Pos1=0.4
[Symbol 91]
Name=R
;S·---·
;|| |
;||·--·
;|| \
;·· E
;POS=X.Y
;-------
Pos1=0.4
Pos2=0.0
Pos3=0.4
Pos4=4.4
Pos5=4.2
Pos6=0.2
Pos7=3.0
[Symbol 92]
Name=R (Inv)
;E·---·
;|| |
;||·--·
;|| \
;·· S
;POS=X.Y
;-------
Pos1=3.0
Pos2=0.2
Pos3=4.2
Pos4=4.4
Pos5=0.4
Pos6=0.0
Pos7=0.4
[Symbol 93]
Name=R2
;·---·
;| |
;|·--·
;| \
;S E
;POS=X.Y
;-------
Pos1=0.0
Pos2=0.4
Pos3=4.4
Pos4=4.2
Pos5=0.2
Pos6=3.0
[Symbol 94]
Name=R2 (Inv)
;·---·
;| |
;|·--·
;| \
;E S
;POS=X.Y
;-------
Pos1=3.0
Pos2=0.2
Pos3=4.2
Pos4=4.4
Pos5=0.4
Pos6=0.0
[Symbol 95]
Name=S
;·---S
;|
;·---·
; |
;E---·
;POS=X.Y
;-------
Pos1=4.4
Pos2=0.4
Pos3=0.2
Pos4=2.2
Pos5=2.0
Pos6=0.0
[Symbol 96]
Name=S (Inv)
;·---E
;|
;·---·
; |
;S---·
;POS=X.Y
;-------
Pos1=0.0
Pos2=2.0
Pos3=2.2
Pos4=0.2
Pos5=0.4
Pos6=4.4
[Symbol 97]
Name=T
;S---·
; ·-·
; |
; |
; E
;POS=X.Y
;-------
Pos1=0.4
Pos2=4.4
Pos3=2.4
Pos4=2.0
[Symbol 98]
Name=T (Inv)
;E---·
; ·-·
; |
; |
; S
;POS=X.Y
;-------
Pos1=2.0
Pos2=2.4
Pos3=4.4
Pos4=0.4
[Symbol 99]
Name=T2
;·---S
;·-·
; |
; |
; E
;POS=X.Y
;-------
Pos1=4.4
Pos2=0.4
Pos3=2.4
Pos4=2.0
[Symbol 100]
Name=T2 (Inv)
;·---E
;·-·
; |
; |
; S
;POS=X.Y
;-------
Pos1=2.0
Pos2=2.4
Pos3=0.4
Pos4=4.4
[Symbol 101]
Name=T3
;·----E
;·--·
; S|
; ||
; ··
;POS=X.Y
;-------
Pos1=2.4
Pos2=2.0
Pos3=2.4
Pos4=0.4
Pos5=4.4
[Symbol 102]
Name=T3 (Inv)
;·----S
;·--·
; E|
; ||
; ··
;POS=X.Y
;-------
Pos1=4.4
Pos2=0.4
Pos3=2.4
Pos4=2.0
Pos5=2.4
[Symbol 103]
Name=T4
;--------E
; \ S
; \ |
; \|
; ·
;POS=X.Y
;-------
Pos1=2.4
Pos2=2.0
Pos3=0.4
Pos4=4.4
[Symbol 104]
Name=T4 (Inv)
;--------S
; \ E
; \ |
; \|
; ·
;POS=X.Y
;-------
Pos1=4.4
Pos2=0.4
Pos3=2.0
Pos4=2.4
[Symbol 105]
Name=U
;S E
;| |
;| |
;| |
;·---·
;POS=X.Y
;-------
Pos1=0.4
Pos2=0.0
Pos3=4.0
Pos4=4.4
[Symbol 106]
Name=U (Inv)
;E S
;| |
;| |
;| |
;·---·
;POS=X.Y
;-------
Pos1=4.4
Pos2=4.0
Pos3=0.0
Pos4=0.4
[Symbol 107]
Name=V
;S E
; \ /
; \ /
; \ /
; ·
;POS=X.Y
;-------
Pos1=0.4
Pos2=2.0
Pos3=4.4
[Symbol 108]
Name=V (Inv)
;E S
; \ /
; \ /
; \/
;POS=X.Y
;-------
Pos1=4.4
Pos2=2.0
Pos3=0.4
[Symbol 109]
Name=W
;S E
; \ /
; \ /\ /
; \/ \/
;POS=X.Y
;-------
Pos1=0.4
Pos2=1.0
Pos3=2.2
Pos4=3.0
Pos5=4.4
[Symbol 110]
Name=W (Inv)
;E S
; \ /
; \ /\ /
; \/ \/
;POS=X.Y
;-------
Pos5=4.4
Pos4=3.0
Pos3=2.2
Pos2=1.0
Pos1=0.4
[Symbol 111]
Name=X
;· S
;|\ /
;| X
;|/ \
;· E
;POS=X.Y
;-------
Pos1=4.4
Pos2=0.0
Pos3=0.4
Pos4=4.0
[Symbol 112]
Name=X (Inv)
;· E
;|\ /
;| X
;|/ \
;· S
;POS=X.Y
;-------
Pos1=4.0
Pos2=0.4
Pos3=0.0
Pos4=4.4
[Symbol 113]
Name=X2
;S ··
; \ //
; ·/·
; // \
;·· E
;POS=X.Y
;-------
Pos1=0.4
Pos2=2.2
Pos3=0.0
Pos4=4.4
Pos5=2.2
Pos6=4.0
[Symbol 114]
Name=X2 (Inv)
;E ··
; \ //
; ·/·
; // \
;·· S
;POS=X.Y
;-------
Pos1=4.0
Pos2=2.2
Pos3=4.4
Pos4=0.0
Pos5=2.2
Pos6=0.4
[Symbol 115]
Name=Y
;S ··
; \ //
; ·/
; /
; E
;POS=X.Y
;-------
Pos1=0.4
Pos2=2.2
Pos3=4.4
Pos4=0.0
[Symbol 116]
Name=Y (Inv)
;E ··
; \ //
; ·/
; /
; S
;POS=X.Y
;-------
Pos1=0.0
Pos2=4.4
Pos3=2.2
Pos4=0.4
[Symbol 117]
Name=Y2
;S ··
; \ //
; ·/
; ·
; |
; E
;POS=X.Y
;-------
Pos1=0.4
Pos2=2.2
Pos3=4.4
Pos4=2.2
Pos5=2.0
[Symbol 118]
Name=Y2 (Inv)
;E ··
; \ //
; ·/
; ·
; |
; S
;POS=X.Y
;-------
Pos1=2.0
Pos2=2.2
Pos3=4.4
Pos4=2.2
Pos5=0.4
[Symbol 119]
Name=Z
;S----·
; /
; /
; /
; /
;·----E
;POS=X.Y
;-------
Pos1=0.4
Pos2=4.4
Pos3=0.0
Pos4=4.0
[Symbol 120]
Name=Z (Inv)
;E----·
; /
; /
; /
; /
;·----S
;POS=X.Y
;-------
Pos1=4.0
Pos2=0.0
Pos3=4.4
Pos4=0.4
/* Misc. */
[Symbol 121]
Name=?
;·---·
;| ·
;S /
; ·
; |
; E
;
;POS=X.Y
;-------
Pos1=0.2
Pos2=0.4
Pos3=4.4
Pos4=4.3
Pos5=2.2
Pos6=2.0
[Symbol 122]
Name=? (Inv)
;·---·
;| ·
;E /
; ·
; |
; S
;
;POS=X.Y
;-------
Pos1=2.0
Pos2=2.2
Pos3=4.3
Pos4=4.4
Pos5=0.4
Pos6=0.2
[Symbol 123]
Name=Delta
; ·
; / \
; / \
; / \
;S E-----·
;
;POS=X.Y
;-------
Pos1=0.0
Pos2=2.4
Pos3=4.0
Pos4=0.0
[Symbol 124]
Name=Delta (Inv)
; ·
; / \
; / \
; / \
;E S-----·
;
;POS=X.Y
;-------
Pos1=0.0
Pos2=4.0
Pos3=2.4
Pos4=0.0
[Symbol 125]
Name=Phi
;S ·-·
;| | |
;·-+-·
; |
; E
;
;POS=X.Y
;-------
Pos1=0.4
Pos2=0.2
Pos3=4.2
Pos4=4.4
Pos5=2.4
Pos6=2.0
[Symbol 126]
Name=Phi (Inv)
;E ·-·
;| | |
;·-+-·
; |
; S
;
;POS=X.Y
;-------
Pos1=2.0
Pos2=2.4
Pos3=4.4
Pos4=4.2
Pos5=0.2
Pos6=0.4<file_sep>-- ahk.lua
-- =======
-- Part of SciTE4AutoHotkey
-- This file implements features specific to AHK in SciTE
-- March 1, 2009 - fincs
-- Functions:
-- AutoIndent
-- Indentation checker and fixer
-- No AutoComplete on comment/string
-- ============================== --
-- Define AutoHotkey lexer styles --
-- ============================== --
local SCE_AHK_DEFAULT = 0
local SCE_AHK_COMMENTLINE = 1
local SCE_AHK_COMMENTBLOCK = 2
local SCE_AHK_ESCAPE = 3
local SCE_AHK_SYNOPERATOR = 4
local SCE_AHK_EXPOPERATOR = 5
local SCE_AHK_STRING = 6
local SCE_AHK_NUMBER = 7
local SCE_AHK_IDENTIFIER = 8
local SCE_AHK_VARREF = 9
local SCE_AHK_LABEL = 10
local SCE_AHK_WORD_CF = 11
local SCE_AHK_WORD_CMD = 12
local SCE_AHK_WORD_FN = 13
local SCE_AHK_WORD_DIR = 14
local SCE_AHK_WORD_KB = 15
local SCE_AHK_WORD_VAR = 16
local SCE_AHK_WORD_SP = 17
local SCE_AHK_WORD_UD = 18
local SCE_AHK_VARREFKW = 19
local SCE_AHK_ERROR = 20
-- ============================================ --
-- OnChar event -- needed to execute AutoIndent --
-- ============================================ --
function OnChar(curChar)
-- this script only works on AHK lexer
-- check for new line
if curChar == "\n" then
-- then execute AutoIndent
AutoIndent()
end
-- disable autocomplete on comment and string
local curStyle = editor.StyleAt[editor.CurrentPos-2]
if ((curStyle == SCE_AHK_COMMENTLINE) or (curStyle == SCE_AHK_COMMENTBLOCK) or (curStyle == SCE_AHK_STRING) or (curStyle == SCE_AHK_ERROR)) then
if editor:AutoCActive() then
editor:AutoCCancel()
end
return true
end
return false
end
-- =================== --
-- AutoIndent function --
-- =================== --
-- this function implements AutoIndent for AutoHotkey
function AutoIndent()
-- get some info
local prevPos = editor:LineFromPosition(editor.CurrentPos) - 1
local prevLine = editor:GetLine(prevPos)
local curPos = prevPos + 1
local curLine = editor:GetLine(curPos)
-- check for comments
if string.find(prevLine, "^%s*;") ~= nil then
return false
end
-- check if it's neccesary to deindent previous line
if (string.find(prevLine, "^%s*}") ~= nil) then
-- deindent the line only if there are tabs
if (string.find(prevLine, "^%s+") ~= nil) then
-- deindent previous line
editor.CurrentPos = editor:PositionFromLine(prevPos)
editor:Home()
editor:BackTab()
-- and deindent current line
editor.CurrentPos = editor:PositionFromLine(curPos)
editor:Home()
editor:BackTab()
end
end
-- check if it's neccesary to indent current line
if ((string.find(prevLine, "^%s*{") ~= nil) or (string.find(prevLine, "{%s*$") ~= nil)) then
-- yep
editor.CurrentPos = editor:PositionFromLine(curPos)
editor:LineEnd()
editor:Tab()
end
-- go to current line
editor.CurrentPos = editor:PositionFromLine(curPos)
editor:LineEnd()
return false
end
-- ==================================== --
-- Indentation checker & fixer function --
-- ==================================== --
-- this function checks the indentation of the script and if neccesary fixes it
function IndentationChecker()
local tabs = 0
local lineIndent = 0
local curLine = ""
-- loop for all lines
local i = 0
while true do
i = i + 1
-- get current line
curLine = editor:GetLine(i)
if curLine == nil then
break
end
-- check for comments
if string.find(curLine, "^%s*;") == nil then
-- go to line
editor.CurrentPos = editor:PositionFromLine(i)
editor:Home()
-- get line indentation
lineIndent = editor.LineIndentation[i]
-- deindent line if neccesary
if lineIndent ~= 0 then
for j = 1, lineIndent, 1 do
editor:BackTab()
end
end
-- indent line if neccesary
if tabs ~= 0 then
for j = 1, tabs, 1 do
editor:Tab()
end
end
-- and fix indentation
-- indent "next" line if is neccesary
if ((string.find(curLine, "^%s*{") ~= nil) or (string.find(curLine, "{%s*$") ~= nil)) then
tabs = tabs + 1
end
-- deindent current line if is neccesary
if (string.find(curLine, "^%s*}") ~= nil) then
editor:Home()
if (string.find(curLine, "%s-.*") ~= nil) then
tabs = tabs - 1
editor:BackTab()
end
end
end
-- go to end of line
editor:LineEnd()
end
end
-- ====================== --
-- Script Backup Function --
-- ====================== --
-- this functions creates backups for the files
function OnBeforeSave(filename)
local backupAllowed = props['make.backup']
if backupAllowed == "1" then
os.remove(filename .. ".bak")
os.rename(filename, filename .. ".bak")
end
end
-- ============ --
-- Open Include --
-- ============ --
function OpenInclude()
local CurrentLine = editor:GetLine(editor:LineFromPosition(editor.CurrentPos))
if not string.find(CurrentLine, "^%s*%#[Ii][Nn][Cc][Ll][Uu][Dd][Ee]") then
print("Not an include line!")
return true
end
local place = string.find(CurrentLine, "%#[Ii][Nn][Cc][Ll][Uu][Dd][Ee]")
local IncFile = string.sub(CurrentLine, place + 8)
if string.find(IncFile, "^[Aa][Gg][Aa][Ii][Nn]") then
IncFile = string.sub(IncFile, 6)
end
IncFile = string.gsub(IncFile, "\r", "") -- strip CR
IncFile = string.gsub(IncFile, "\n", "") -- strip LF
IncFile = string.sub(IncFile, 2) -- strip space at the beginning
IncFile = string.gsub(IncFile, "*i ", "") -- strip *i option
IncFile = string.gsub(IncFile, "*I ", "")
-- Delete comments
local cplace = string.find(IncFile, ";")
if cplace then
IncFile = string.sub(IncFile, 1, cplace-1)
end
-- Delete spaces at the beginning if needed
while true do
ccc = string.find(IncFile, "^%s")
if ccc then
IncFile = string.sub(IncFile, ccc+1)
else
break
end
end
-- Delete spaces at the end if needed
while true do
ccc = string.find(IncFile, "%s$")
if ccc then
IncFile = string.sub(IncFile, 1, ccc-1)
else
break
end
end
-- print("Include: '" .. IncFile .. "'")
if io.open(IncFile, "r") then
io.close()
scite.Open(IncFile)
else
print("File not found! Specified: '" .. IncFile .. "'")
end
return true
end
-- ================ --
-- Helper Functions --
-- ================ --
<file_sep>[Favorites]
Griffin Labs Projects=C:\Documents and Settings\Scotty\My Documents\Griffin Labs Projects
My Tools 2005=C:\Documents and Settings\Scotty\My Documents\Visual Studio 2005\Projects\My Tools
My Tools 2008=C:\Documents and Settings\Scotty\My Documents\Visual Studio 2008\Projects\My Tools
Program Files=C:\Program Files
Y Drive - Scott Rippey=\\Intel-giga\public--y\Scott Rippey
| 3c3569b0e7800836fc97e6dad8733c6b6c5f6042 | [
"Text",
"INI",
"Lua"
] | 7 | INI | scottrippey/ScottoHotKey | 6479c324ad6ed1a832db588d2745f2e53a4efca5 | 5ee45c8741d4a517a1a635c729923783b26ccc2c | |
refs/heads/main | <file_sep>-- phpMyAdmin SQL Dump
-- version 5.0.3
-- https://www.phpmyadmin.net/
--
-- Host: 127.0.0.1
-- Generation Time: May 07, 2021 at 02:24 PM
-- Server version: 10.4.14-MariaDB
-- PHP Version: 7.2.34
SET SQL_MODE = "NO_AUTO_VALUE_ON_ZERO";
START TRANSACTION;
SET time_zone = "+00:00";
/*!40101 SET @OLD_CHARACTER_SET_CLIENT=@@CHARACTER_SET_CLIENT */;
/*!40101 SET @OLD_CHARACTER_SET_RESULTS=@@CHARACTER_SET_RESULTS */;
/*!40101 SET @OLD_COLLATION_CONNECTION=@@COLLATION_CONNECTION */;
/*!40101 SET NAMES utf8mb4 */;
--
-- Database: `prpl`
--
-- --------------------------------------------------------
--
-- Table structure for table `produk`
--
CREATE TABLE `produk` (
`id` int(6) NOT NULL,
`nama_produk` varchar(30) NOT NULL,
`harga` int(50) NOT NULL,
`tipe_produk` varchar(30) NOT NULL,
`stok` int(50) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
--
-- Dumping data for table `produk`
--
INSERT INTO `produk` (`id`, `nama_produk`, `harga`, `tipe_produk`, `stok`) VALUES
(3, 'Super 25', 35000, 'Baju', 16),
(5, 'Uyta', 117000, 'Celana', 34),
(6, '<NAME>', 235000, 'Celana', 13),
(7, 'Vander', 125000, 'Celana', 42),
(8, 'Super 25', 35000, 'Baju', 16),
(10, 'Uyta', 117000, 'Celana', 34);
--
-- Indexes for dumped tables
--
--
-- Indexes for table `produk`
--
ALTER TABLE `produk`
ADD PRIMARY KEY (`id`);
--
-- AUTO_INCREMENT for dumped tables
--
--
-- AUTO_INCREMENT for table `produk`
--
ALTER TABLE `produk`
MODIFY `id` int(6) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=11;
COMMIT;
/*!40101 SET CHARACTER_SET_CLIENT=@OLD_CHARACTER_SET_CLIENT */;
/*!40101 SET CHARACTER_SET_RESULTS=@OLD_CHARACTER_SET_RESULTS */;
/*!40101 SET COLLATION_CONNECTION=@OLD_COLLATION_CONNECTION */;
<file_sep>-- phpMyAdmin SQL Dump
-- version 5.0.3
-- https://www.phpmyadmin.net/
--
-- Host: 127.0.0.1
-- Generation Time: May 07, 2021 at 02:25 PM
-- Server version: 10.4.14-MariaDB
-- PHP Version: 7.2.34
SET SQL_MODE = "NO_AUTO_VALUE_ON_ZERO";
START TRANSACTION;
SET time_zone = "+00:00";
/*!40101 SET @OLD_CHARACTER_SET_CLIENT=@@CHARACTER_SET_CLIENT */;
/*!40101 SET @OLD_CHARACTER_SET_RESULTS=@@CHARACTER_SET_RESULTS */;
/*!40101 SET @OLD_COLLATION_CONNECTION=@@COLLATION_CONNECTION */;
/*!40101 SET NAMES utf8mb4 */;
--
-- Database: `login`
--
-- --------------------------------------------------------
--
-- Table structure for table `users`
--
CREATE TABLE `users` (
`id_users` int(11) NOT NULL,
`username` varchar(50) COLLATE utf8mb4_unicode_ci DEFAULT NULL,
`password` varchar(100) COLLATE utf8mb4_unicode_ci DEFAULT NULL,
`nama` varchar(100) COLLATE utf8mb4_unicode_ci DEFAULT NULL,
`email` varchar(200) COLLATE utf8mb4_unicode_ci DEFAULT NULL,
`no_hp` varchar(200) COLLATE utf8mb4_unicode_ci DEFAULT NULL,
`token` varchar(255) COLLATE utf8mb4_unicode_ci DEFAULT NULL,
`aktif` enum('1','0') COLLATE utf8mb4_unicode_ci DEFAULT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_unicode_ci;
--
-- Dumping data for table `users`
--
INSERT INTO `users` (`id_users`, `username`, `password`, `nama`, `email`, `no_hp`, `token`, `aktif`) VALUES
(17, 'Laraspakaya', '<PASSWORD>', '<NAME>', '<EMAIL>', '08778932901', '76a29d45a7499f1d6edc1a0b373669e75b4474e83000d12e771730e628a69aa4', '0');
--
-- Indexes for dumped tables
--
--
-- Indexes for table `users`
--
ALTER TABLE `users`
ADD PRIMARY KEY (`id_users`);
--
-- AUTO_INCREMENT for dumped tables
--
--
-- AUTO_INCREMENT for table `users`
--
ALTER TABLE `users`
MODIFY `id_users` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=18;
COMMIT;
/*!40101 SET CHARACTER_SET_CLIENT=@OLD_CHARACTER_SET_CLIENT */;
/*!40101 SET CHARACTER_SET_RESULTS=@OLD_CHARACTER_SET_RESULTS */;
/*!40101 SET COLLATION_CONNECTION=@OLD_COLLATION_CONNECTION */;
| 31b5c65f742b767580fde36fa6d1ca34780f7fc9 | [
"SQL"
] | 2 | SQL | laraspakaya/prpl2021 | 89758e9ea4de65425aa3b01b941ee05f6c32821b | f7768b523791b35e3d80de1beebf3158c5989f3f | |
refs/heads/master | <repo_name>Cafemug/JDBC<file_sep>/src/hotel/gui2.java
package hotel;
import java.awt.Choice;
import java.awt.Color;
import java.awt.Component;
import java.awt.Font;
import java.awt.GridLayout;
import java.awt.TextField;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.ItemEvent;
import java.awt.event.ItemListener;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.io.File;
import java.io.FileNotFoundException;
import java.sql.SQLException;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Calendar;
import java.util.Date;
import java.util.Scanner;
import java.util.StringTokenizer;
import javax.swing.JButton;
import javax.swing.JComboBox;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JMenuItem;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTabbedPane;
import javax.swing.JTextArea;
import javax.swing.JTextField;
import javax.swing.ScrollPaneConstants;
import javax.swing.border.LineBorder;
import javax.swing.border.SoftBevelBorder;
import hotel.dbconnection;
class gui2 implements ActionListener, ItemListener {
static dbconnection dbcon = new dbconnection();
//직원 로그인시 그 직원의 이름과 권한을 받아두는 변수입니다.
static String staffname = ""; static String stafflevel = "";
JFrame frame = new JFrame();
//open메뉴에 들어갈 라벨들
JMenuBar upmenu;
JMenuItem fileopen, menulogin;/////
JFileChooser jf = new JFileChooser();
JLabel NameLabel_menu,StaffName,date,GuestNamelabel,title,room,roomname,stateroom,menu,regi,currentdate;
JLabel customer,year,ro, day;
static JPanel ptable,search_regi,personTab, RoomTab,StaffTab,menuTab,reserve_panel;
JTextField personname_T;
TextField Nametext_menu,insertcustomer,insertyear;
TextField StaffNametext;
TextField GuestNametext;
static JTextArea TextArea,textarea_room,Stafftextarea,textarea_menu;/////주문내역화면
static Choice tableNumber,datechoice,roomnum,daycount,roomno;
JButton StaffRegi,StaffSearch,menuRegi_menu,Searchmenu;
JButton GuestRegi,GuestSearch, enroll,cancel;
JTabbedPane tabPanel;
static JLabel table[] = new JLabel[21];
public gui2() throws SQLException, ParseException{
frame = new JFrame("HOTEL");// 프레임 제목
frame.setLayout(null); //상당 메뉴바 생성
upmenu = new JMenuBar(); //상당 메뉴바 생성
//visible
JMenu file = new JMenu("file"); //메뉴바 상위메뉴
fileopen = new JMenuItem("Open"); //메뉴바 하위메뉴 생성
file.add(fileopen); //상위메뉴에 하위메뉴 생성
upmenu.add(file); //상위메뉴를 메뉴바에 추가
fileopen.addActionListener((ActionListener) this);
frame.setJMenuBar(upmenu); //visible
//이곳엔 호텔 관리시스템, 투숙예약, 객실예약 현황, 현재 날짜 , 등록/조회 라벨 생성
/////title Label
title = new JLabel ("호텔 관리 시스템",JLabel.CENTER);
title.setFont(new Font("돋움",Font.BOLD,25)); //글씨체
title.setBounds(90,10,500,50); //label 위치
title.setBorder(new LineBorder(Color.black)); // label 테두리설정
room = new JLabel("객실 예약현황",JLabel.CENTER);
room.setFont(new Font("돋움",Font.BOLD,15));
room.setBounds(320,65,150,20);
Calendar cDateCal = Calendar.getInstance();
cDateCal.add(Calendar.DATE, 0);
SimpleDateFormat sdf = new SimpleDateFormat("yyyy/MM/dd");
String result = sdf.format(cDateCal.getTime());
currentdate = new JLabel(result,JLabel.RIGHT);
currentdate.setFont(new Font("돋움",Font.BOLD,15));
currentdate.setBounds(520,65,150,20);
stateroom = new JLabel("투숙내역",JLabel.CENTER);
stateroom.setFont(new Font("돋움",Font.BOLD,15));
stateroom.setBounds(20,65,60,20);
regi = new JLabel("등록/조회",JLabel.CENTER);
regi.setFont(new Font("돋움",Font.BOLD,15));
regi.setBounds(20,330,80,22);
reserve_panel = new JPanel();
reserve_panel.setLayout(null);
customer = new JLabel("고객명");
customer.setBounds(30,10,120,30);
insertcustomer = new TextField();
insertcustomer.setBounds(160,10,120,30);
year = new JLabel("체크인(YYYYMMDD)");
year.setBounds(30,50,120,30);
insertyear= new TextField();
insertyear.setBounds(160,50,120,30);
day = new JLabel("박");
day.setBounds(30,90,120,30);
daycount = new Choice();
for(int i=1;i<=10;i++) {
String idx=String.valueOf(i);
daycount.add(idx);
}
daycount.addItemListener(this);
daycount.setBounds(160,90,120,40);
ro = new JLabel("객실");
ro.setBounds(30,130,120,30);
roomnum = new Choice();
dbcon.currentroomno();
roomnum.addItemListener(this);
roomnum.setBounds(160,130,120,40);
enroll = new JButton("예약 등록/변경");
enroll.addActionListener(this);
enroll.setFont(new Font("돋움",Font.BOLD,11));
enroll.setBounds(30,160,120,60);
cancel = new JButton("예약 취소");
cancel.addActionListener(this);
cancel.setBounds(160,160,120,60);
cancel.setFont(new Font("돋움",Font.BOLD,11));
reserve_panel.add(customer);
reserve_panel.add(insertcustomer);
reserve_panel.add(year);
reserve_panel.add(insertyear);
reserve_panel.add(day);
reserve_panel.add(daycount);
reserve_panel.add(ro);
reserve_panel.add(roomnum);
reserve_panel.add(enroll);
reserve_panel.add(cancel);
reserve_panel.setBounds(20,85,300,230);
frame.add(reserve_panel);
//테이블 현황 패널 생성
ptable = new JPanel(new GridLayout(4,5,5,5));
for(int i=1; i<=10; i++){
table[i] = new JLabel(100+i+"");
table[i].setFont(new Font("돋움",Font.BOLD,15));
table[i].setBorder(new LineBorder(Color.black)); //모든 라벨의 테두리에 검은 선이 보이게 추가
table[i].setOpaque(true); //라벨의 배경색을 보이게 해주는 것
table[i].setBackground(Color.WHITE); // 배경색을 흰색으로 통일
table[i].setHorizontalAlignment(JLabel.CENTER); //라벨안의 호수 숫자를 가운데 정렬
ptable.add(table[i]); // 패널에 라벨을 집어넣음
}
for(int i=11; i<=20; i++){
table[i] = new JLabel(200+i-10+"");
table[i].setFont(new Font("돋움",Font.BOLD,15));
table[i].setBorder(new LineBorder(Color.black)); //모든 라벨의 테두리에 검은 선이 보이게 추가
table[i].setOpaque(true); //라벨의 배경색을 보이게 해주는 것
table[i].setBackground(Color.WHITE); // 배경색을 흰색으로 통일
table[i].setHorizontalAlignment(JLabel.CENTER); //라벨안의 호수 숫자를 가운데 정렬
ptable.add(table[i]); // 패널에 라벨을 집어넣음
}
ptable.setBounds(340, 90, 330, 220);/////
ptable.setBorder(new LineBorder(Color.black));
search_regi = new JPanel();
search_regi.setLayout(null);
tabPanel = new JTabbedPane();
tabPanel.setBounds(10, 0, 610, 295);
personTab = new JPanel();
personTab.setBorder(new LineBorder(Color.black));
personTab.setLayout(null);
GuestNamelabel = new JLabel("고객명");
GuestNamelabel.setFont(new Font("돋움",Font.BOLD,13));
GuestNamelabel.setBounds(20,40,60,20);
GuestNametext = new TextField(10);
GuestNametext.setBounds(80,40,80,25);
GuestRegi = new JButton("가입");
GuestRegi.addActionListener(this);
GuestRegi.setBounds(25,80,55,30);
GuestRegi.setFont(new Font("돋움", Font.PLAIN, 10));
GuestSearch = new JButton("조회");
GuestSearch.setFont(new Font("돋움", Font.PLAIN, 10));
GuestSearch.addActionListener(this);
GuestSearch.setBounds(95,80,55,30);
TextArea = new JTextArea(10,20);
TextArea.setBounds(250,40,300,180);
TextArea.setBorder(new LineBorder(Color.black));
TextArea.setEditable(false);
personTab.add(GuestNamelabel);
personTab.add(GuestNametext);
personTab.add(GuestRegi);
personTab.add(GuestSearch);
personTab.add(TextArea);
RoomTab = new JPanel();
RoomTab.setLayout(null);
RoomTab.setBorder(new LineBorder(Color.black));
roomname=new JLabel("객실");
roomname.setFont(new Font("돋움",Font.BOLD,15));
roomname.setBounds(20,40,60,20);
roomno = new Choice();
dbcon.getroomno();
roomno.setBounds(80,40,80,25);
roomno.addItemListener(this);
textarea_room = new JTextArea(10,20);
textarea_room.setBounds(250,40,300,180);/////
textarea_room.setBorder(new LineBorder(Color.black));
textarea_room.setEditable(false);
RoomTab.add(textarea_room);
RoomTab.add(roomname);
RoomTab.add(roomno);
StaffTab = new JPanel();
StaffTab.setLayout(null);
StaffTab.setBorder(new LineBorder(Color.black));
StaffName = new JLabel("직원명");
StaffName.setFont(new Font("돋움",Font.BOLD,15));
StaffName.setBounds(20,40,60,20);
StaffNametext = new TextField(10);
StaffNametext.setBounds(80,40,80,25);
StaffRegi = new JButton("직원등록");
StaffRegi.addActionListener(this);
StaffRegi.setBounds(25,80,75,30);
StaffRegi.setFont(new Font("돋움", Font.PLAIN, 10));
StaffSearch = new JButton("조회");
StaffSearch.addActionListener(this);
StaffSearch.setBounds(115,80,55,30);
StaffSearch.setFont(new Font("돋움", Font.PLAIN, 10));
Stafftextarea = new JTextArea(10,20);
Stafftextarea.setBounds(250,40,300,180);
Stafftextarea.setBorder(new LineBorder(Color.black));
Stafftextarea.setEditable(false);
StaffTab.add(StaffNametext);
StaffTab.add(StaffName);
StaffTab.add(StaffNametext);
StaffTab.add(StaffRegi);
StaffTab.add(StaffSearch);
StaffTab.add(Stafftextarea);
tabPanel.add("고객",personTab);
tabPanel.add("객실",RoomTab);
tabPanel.add("직원",StaffTab);
search_regi.add(tabPanel);
search_regi.setBounds(25, 355, 630, 300);
search_regi.setBorder(new LineBorder(Color.black));
frame.add(title);
frame.add(room);
frame.add(stateroom);
frame.add(regi);
frame.add(ptable);
frame.add(search_regi);
frame.add(currentdate);
frame.setSize(700,730);
frame.setLocation(0,0);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
dbcon.updatetable();
}
public void readFile(File textfile) throws SQLException{
Scanner scanner = null;
int indexcount = 0;
int check = 0;
try {
scanner = new Scanner(textfile);
while(scanner.hasNextLine()){
String test = scanner.nextLine().toString();
try{
int number = Integer.parseInt(test);
check ++;
if(check == 1){
System.out.println("회원목록입니다");
while(indexcount < number){
test = scanner.nextLine().toString();
StringTokenizer token = new StringTokenizer(test,"\t");
while(token.hasMoreTokens()){
String cname = token.nextToken();
String cgender = token.nextToken();
String caddress = token.nextToken();
String cphone = token.nextToken();
System.out.println("guestname = " + cname + ", guestID = " + cgender+", guestBirthday = " + caddress+", guestLevel = " + cphone);
dbcon.regicustomer(cname, cgender, caddress, cphone);
}
indexcount ++ ;
}
indexcount = 0;
}
else if(check == 2){
while(indexcount < number){
test = scanner.nextLine().toString();
StringTokenizer token = new StringTokenizer(test,"\t");
while(token.hasMoreTokens()){
String mname = token.nextToken();
String mgender = token.nextToken();
String maddress = token.nextToken();
String mphone = token.nextToken();
dbcon.regimanager(mname, mgender,maddress, mphone);
}
indexcount ++ ;
}
indexcount = 0;
}
else if(check == 3){
while(indexcount < number){
test = scanner.nextLine().toString();
StringTokenizer token = new StringTokenizer(test,"\t");
while(token.hasMoreTokens()){
int roomno = Integer.parseInt(token.nextToken());
int ableno = Integer.parseInt(token.nextToken());
String roomtype = token.nextToken();
dbcon.regiroom(roomno,ableno,roomtype);
}
indexcount ++ ;
}
indexcount = 0;
}
}
catch(Exception e){
JOptionPane.showMessageDialog(null, "파일 읽기에 실패했습니다. : 다시 시도해 주세요" );
}
}
}
catch (FileNotFoundException e) {
JOptionPane.showMessageDialog(null, "파일 읽기에 실패했습니다. : 다시 시도해 주세요" );
}
catch (Exception e) {
JOptionPane.showMessageDialog(null, "파일 읽기에 실패했습니다. : 다시 시도해 주세요" );
}
finally{
scanner.close();
}
}
public void itemStateChanged(ItemEvent e) {
if(e.getSource() == roomno){
try {
dbcon.getroom(roomno.getSelectedItem());
} catch (SQLException e1) {
e1.printStackTrace();
}
}
}
public void actionPerformed(ActionEvent e) {
if(e.getSource()== fileopen){
if(jf.showOpenDialog(upmenu) == JFileChooser.APPROVE_OPTION){
File fSelectedFile = jf.getSelectedFile();
try {
readFile(fSelectedFile);
} catch (Exception e1) {
JOptionPane.showMessageDialog(null, "파일을 읽어들이는 도중 문제가 발생했습니다. 다시 시도해 주세요.");
}
}
}
else if (e.getSource() == enroll){
if(insertcustomer.getText().trim().length() == 0 || insertyear.getText().trim().length() == 0 ){
JOptionPane.showMessageDialog(null, "입력을 다 하지 않았습니다");
}else{
try {
int number = Integer.parseInt(roomnum.getSelectedItem().toString());
dbcon.reservation(insertcustomer.getText().trim(), insertyear.getText().trim() ,Integer.parseInt(daycount.getSelectedItem().toString()),number);
}
catch (NumberFormatException e1) {
JOptionPane.showMessageDialog(null, e1);
} catch (SQLException e1) {
e1.printStackTrace();
}
}
}
else if (e.getSource() == cancel){
if(insertcustomer.getText().trim().length() == 0 || insertyear.getText().trim().length() == 0 ){
JOptionPane.showMessageDialog(null, "입력을 다 하지 않았습니다");
}else{
try {
int number = Integer.parseInt(roomnum.getSelectedItem().toString());
dbcon.modifyres(insertcustomer.getText().trim(), insertyear.getText().trim());
}
catch (NumberFormatException e1) {
JOptionPane.showMessageDialog(null, e1);
} catch (SQLException e1) {
e1.printStackTrace();
}
}
}
else if(e.getSource() == GuestRegi){
regiguest gr = new regiguest();
gr.guestRegistration();
}
else if (e.getSource() == GuestSearch){
if(GuestNametext.getText().trim().length() == 0){
JOptionPane.showMessageDialog(null, "회원이름을 입력해주세요");
}
else{
try{
dbcon.getcustomerInformation(GuestNametext.getText().trim());
}catch(Exception e1){
JOptionPane.showMessageDialog(null, "회원이름을 가져오는 도중에 문제가 발생했습니다."+ e1);
}
}
}
else if (e.getSource() == StaffRegi){
registaff sr = new registaff();
sr.staffRegistration();
}
else if(e.getSource() == StaffSearch){
if(StaffNametext.getText().trim().length() == 0){
JOptionPane.showMessageDialog(null, "직원이름을 입력해주세요");
}
else{
try{
dbcon.getmanagerInformation(StaffNametext.getText().trim());
}catch(SQLException e1){
JOptionPane.showMessageDialog(null, "직원이름을 가져오는 도중에 문제가 발생했습니다."+ e1);
}
}
}
}
}
<file_sep>/README.md
# JDBC
JAVA Swing Hotel reservation management
| c43e521ab89191b17ed3cdbb274b68296e07fedb | [
"Markdown",
"Java"
] | 2 | Java | Cafemug/JDBC | db1bc8ada9714ce2ccb9d19db39ee2d2814f54f7 | 0086d5089000c2c63744656795a043abca2587b0 | |
refs/heads/master | <file_sep>import React, { useState, useEffect } from 'react';
import api from './services/api';
import './App.css';
// import backgroundImage from './assets/background.jpg';
import Header from './components/Header';
function App() {
// const [projects, setProjects] = useState(['Desenvolvimento de app', 'Front-end web']);
const [projects, setProjects] = useState([]);
useEffect(() => {
api.get('projects').then(response => {
setProjects(response.data);
})
}, [])
async function handleAddProject() {
// projects.push(`Novo Projeto ${Date.now()}`); nao faz mais assim
// setProjects([...projects, `Novo Projeto ${Date.now()}`]) //conceito de imutabilidade
const response = await api.post('projects', {
title: `Front-end com VueJS ${Date.now()}`,
owner: "<NAME>"
})
const project = response.data;
setProjects([...projects, project]);
}
return (
<>
<Header title="Projects" />
{/* <img width={500} src={backgroundImage}/> */}
<ul>
{/* {projects.map(project => <li key={project}>{project}</li>)} */}
{projects.map(project => <li key={project.id}>{project.title}</li>)}
</ul>
<button type="button" onClick={handleAddProject}>Adicionar Projeto</button>
{/* <Header title="Bino">
<ul>
<li>petisco</li>
<li>passear</li>
</ul>
</Header>
<Header title="Paula">
<ul>
<li>comer</li>
<li>dormir</li>
</ul>
</Header>
<Header title="Livia">
<ul>
<li>palmeiras</li>
</ul>
</Header> */}
</>
)
}
export default App;
// componente, propriedade e estado (e imutabilidade)
//map -> percorre e retorna
//useState retorna um array com 2 posicoes
//1. Variavel com o seu valor inicial
//2. Funcao para atualizarmos o valor
//useEffect -> dispara funcoes sempre que tivermos alguma info alterada, ou quando componente
//for exibido em tela
//1. Qual funcao quero disparar
//2. Quando quero disparar | 53de402b6a2e7d1ea148eba4334ee876370e94d7 | [
"JavaScript"
] | 1 | JavaScript | paulapompeo/conceitos-dev | 2d41e86c7aaa921ba457b9a47bad02bd67b9506c | aefe55c361dc4800e42311560ebc0bba86f6de33 | |
refs/heads/master | <repo_name>DavidTOUCHARD/Bibliotheque<file_sep>/BibliothequeEnLigne/src/eu/ensup/bibliothequeenligne/dao/ConnectionDao.java
package eu.ensup.bibliothequeenligne.dao;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
import java.sql.Statement;
public class ConnectionDao {
public void connect() {
String url = "jdbc:mysql//localhost/bibliothequeenligne";
String login = "root";
String password = "";
Connection cn = null;
Statement st = null;
try {
Class.forName("com.mysql.jdbc.Driver");
cn = DriverManager.getConnection(url, login, password);
st = cn.createStatement();
String sql = "SELECT * FROM `etudiant`";
st.executeUpdate(sql);
} catch (SQLException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
} finally {
try {
cn.close();
st.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
<file_sep>/BibliothequeEnLigne/src/eu/ensup/bibliothequeenligne/domaine/Livre.java
package eu.ensup.bibliothequeenligne.domaine;
public class Livre {
private String titre;
private String auteur;
private String dateparution;
public String getTitre() {
return titre;
}
public void setTitre(String titre) {
this.titre = titre;
}
public String getAuteur() {
return auteur;
}
public void setAuteur(String auteur) {
this.auteur = auteur;
}
public String getDateparution() {
return dateparution;
}
public void setDateparution(String dateparution) {
this.dateparution = dateparution;
}
@Override
public String toString() {
return "Livre [titre=" + titre + ", auteur=" + auteur + ", dateparution=" + dateparution + "]";
}
public Livre(String titre, String auteur, String dateparution) {
super();
this.titre = titre;
this.auteur = auteur;
this.dateparution = dateparution;
}
}
| 5bf69d6a20a775196bb47aa80f43213f52dfc12d | [
"Java"
] | 2 | Java | DavidTOUCHARD/Bibliotheque | 99ce338bc162a4e9f3fffb36fbc223f522d11771 | 547c3b82035361d8aeed218077d9e2e62813450a | |
refs/heads/master | <repo_name>sojour/boiler-maker<file_sep>/server/db/database.js
const Sequelize = require('sequelize');
//be sure to augment the db name if necessary
const db = new Sequelize(process.env.DATABASE_URL || 'postgres://localhost:5432/boiler-maker', {
logging: false
});
module.exports = db;
| 7262f813dca4ab4087a8f0a5309d40d3aa8b6c66 | [
"JavaScript"
] | 1 | JavaScript | sojour/boiler-maker | 14be8094e3d868430ed0e62c5f6a8b95761356f6 | c202f7c2725260fa5f14f3f7e745fe649b5f3988 | |
refs/heads/main | <file_sep>//
// SDSOrientationAdaptiveStack.swift
//
// Created by : <NAME> on 2021/06/03
// © 2021 SmallDeskSoftware
//
import SwiftUI
import Combine
import UIKit
extension UIDevice {
static public func stableDeviceOrientation() -> UIDeviceOrientation {
if UIDevice.current.orientation.isValidInterfaceOrientation {
return UIDevice.current.orientation
} else {
return UIScreen.main.bounds.size.width > UIScreen.main.bounds.size.height ? UIDeviceOrientation.landscapeLeft : UIDeviceOrientation.portrait
}
}
}
extension UIDeviceOrientation: CustomStringConvertible {
public var description: String {
switch self {
case .unknown:
return "unknown"
case .portrait:
return "portrait"
case .portraitUpsideDown:
return "portraitUpsideDown"
case .landscapeLeft:
return "landscapeLeft"
case .landscapeRight:
return "landscapeRight"
case .faceUp:
return "faceUp"
case .faceDown:
return "faceDown"
default:
return "unknown"
}
}
}
public struct SDSOrientationAdaptiveStack<Content1: View, Content2: View> : View {
var firstContent: Content1
var secondContent: Content2
@State var orientation: UIDeviceOrientation = UIDevice.current.orientation
@Binding var firstContentRatio: CGFloat
public init(@ViewBuilder first: () -> Content1, @ViewBuilder second: () -> Content2, ratio: Binding<CGFloat> = .constant(0.5) ) {
self.firstContent = first()
self.secondContent = second()
self._firstContentRatio = ratio
self._orientation = State(wrappedValue: UIDevice.stableDeviceOrientation())
}
public var body: some View {
ZStack {
GeometryReader { geom in
if orientation == .landscapeRight || orientation == .landscapeLeft {
HStack {
if firstContentRatio > 0.01 {
firstContent
.frame(width: geom.size.width * firstContentRatio)
} else {
EmptyView()
}
if firstContentRatio < 0.99 {
secondContent
.frame(width: geom.size.width * (1.0-firstContentRatio))
} else {
EmptyView()
}
}
} else {
VStack {
if firstContentRatio > 0.01 {
firstContent
.frame(height: geom.size.height * firstContentRatio)
} else {
EmptyView()
}
if firstContentRatio < 0.99 {
secondContent
.frame(height: geom.size.height * (1.0-firstContentRatio))
} else {
EmptyView()
}
}
}
}
}
.onAppear(perform: {
orientation = UIDevice.stableDeviceOrientation()
})
.onReceive(NotificationCenter.default.publisher(for: UIDevice.orientationDidChangeNotification)) { _ in
orientation = UIDevice.stableDeviceOrientation()
}
}
}
<file_sep># SDSOrientationAdaptiveStack



<!--
comment
-->
## Feature
switch HStack / VStack according to device orientation
## in 30 sec.
https://user-images.githubusercontent.com/6419800/120910504-683d5a00-c6ba-11eb-973b-a091a29f4f7b.mp4
## Code Example
```
//
// ContentView.swift
//
// Created by : <NAME> on 2021/06/03
// © 2021 SmallDeskSoftware
//
import SwiftUI
import SDSOrientationAdaptiveStack
struct ContentView: View {
var body: some View {
SDSOrientationAdaptiveStack(first: {
Rectangle()
.fill(Color.red)
.frame(width: 100, height: 100)
.overlay(Text("First"))
}, second: {
Rectangle()
.fill(Color.green)
.frame(width: 100, height: 100)
.overlay(Text("Second"))
})
.padding()
}
}
```
## Installation
use Swift Package Manager: URL: https://github.com/tyagishi/SDSOrientationAdaptiveStack
## Requirements
none
## Note
<file_sep> import XCTest
@testable import SDSOrientationAdaptiveStack
import SwiftUI
import UIKit
final class SDSOrientationAdaptiveStackTests: XCTestCase {
func testLayout() throws {
let sut = ContentView()
XCTAssertNotNil(sut)
}
}
struct ContentView: View {
var body: some View {
SDSOrientationAdaptiveStack(first: {
Rectangle()
.fill(Color.red)
.frame(width: 100, height: 100)
.overlay(Text("First"))
.accessibility(identifier: "firstRect")
}, second: {
Rectangle()
.fill(Color.green)
.frame(width: 100, height: 100)
.overlay(Text("Second"))
})
.padding()
}
}
| 85d66e3442930dc4c2f717c86586bd11a6014225 | [
"Swift",
"Markdown"
] | 3 | Swift | tyagishi/SDSOrientationAdaptiveStack | 75ecefc443dbf6468b4de744bdd77410581a3938 | f62ac25f2c3bc93312757a573516f133680a0498 | |
refs/heads/master | <file_sep>package com.adp.autopay.automation.pagespecificlibrary;
import com.adp.autopay.automation.framework.CustomWebElement;
public class Popup extends CustomWebElement
{
}<file_sep>package com.adp.autopay.automation.cucumberRunner;
import com.adp.autopay.automation.commonlibrary.DataHelper;
import com.adp.autopay.automation.mongodb.MongoDB;
import com.adp.autopay.automation.utility.*;
import cucumber.runtime.java.ObjectContainer;
import org.openqa.selenium.Capabilities;
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.Platform;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.ie.InternetExplorerDriver;
import org.openqa.selenium.remote.CapabilityType;
import org.openqa.selenium.remote.DesiredCapabilities;
import org.openqa.selenium.remote.RemoteWebDriver;
import org.testng.ITestContext;
import org.testng.annotations.*;
import java.io.File;
import java.net.InetAddress;
import java.net.URL;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
import java.util.concurrent.TimeUnit;
/**
* An example of using TestNG when the test class does not inherit from
* AbstractTestNGCucumberTests.
*/
//@CucumberOptions(plugin = {"html:target/cucumber-html-report"},features={"src/main/java/com/adp/aca/automation/features/production/US125150_TC97499_Home_RequireInformation.feature"},
// glue = {"com.adp.aca.automation.teststeps","com.adp.aca.automation.cucumberRunner"})
public class RunCukesByCompositionTest extends RunCukes{
public static String SuiteName;
public ExecutionContext context;
public String FeatureName;
private MongoDB mongoDB;
public String strTestRunId;
public ITestContext TestContext;
public String SRunAgainst;
public String MachineIP;
public String browserVersion;
public static String SuiteRunId;
public static String mailList="NoMailList";
public List<HashMap<String,String>> datamap;
//public List<String> ignoreTags ;
/* ********************************** Before Suite ********************************* */
@BeforeSuite
public void beforeSuite(ITestContext istx) throws Exception {
try {
System.out.println(istx);
istx.getSuite().getParallel();
SuiteContext.SuiteRunId = GenerateKey.GenerateExecutionId("SUITE");
SuiteContext.StartTime = new Date(System.currentTimeMillis());
SuiteRunId = SuiteContext.SuiteRunId;
SuiteContext.SuiteName = istx.getSuite().getName();
SuiteName = istx.getSuite().getName();
istx.getSuite().getParameter("mailList");
MongoDB mongoDB = new MongoDB();
mongoDB.connectMongoDB();
mongoDB.startSuiteExecution(SuiteContext.SuiteRunId, SuiteName);
mongoDB.disconnectMongoDB();
System.out.println("Before Suite");
} catch (Exception e) {
System.out.println("");
}
}
/* *********************************** Before Test ***************************** */
@Parameters({"FeatureName","RunAgainst","Client","platform","browser","version"})
@BeforeTest
public void beforeTest(ITestContext ctx,String FeatureName,String RunAgainst,String Client,String platform, String browser, String version) throws Exception {
try {
SuiteName = ctx.getSuite().getName();
System.out.println("Before Test Method");
ObjectContainer.addClass(ExecutionContext.class);
this.context = ObjectContainer.getInstance(ExecutionContext.class);
Date date = new Date(System.currentTimeMillis());
this.context.StartTime = date;
this.context.SuiteRunId = this.SuiteRunId;
this.context.SuiteName = SuiteName;
MongoDB mongoDB = new MongoDB();
mongoDB.connectMongoDB();
//Configuration starts Here
SRunAgainst = ctx.getCurrentXmlTest().getParameter("RunAgainst");
if(mailList=="NoMailList" || mailList==null||mailList=="")
mailList=ctx.getCurrentXmlTest().getParameter("mailList");
this.context.FeatureName = ctx.getCurrentXmlTest().getParameter("FeatureName");
this.context.browser = ctx.getCurrentXmlTest().getParameter("browser");
this.context.platform = ctx.getCurrentXmlTest().getParameter("platform");
this.context.version = ctx.getCurrentXmlTest().getParameter("version");
this.context.InputDataFilePath = ctx.getCurrentXmlTest().getParameter("InputDataFilePath");
this.context.ServicesDataFilePath = ctx.getCurrentXmlTest().getParameter("ServicesDataFilePath");
//this.context.InputDataSheet = ctx.getCurrentXmlTest().getParameter("InputDataSheet");
this.context.ServicesDataSheet = ctx.getCurrentXmlTest().getParameter("ServicesDataSheet");
//this.context.ignoreTags=ignoreTags;
//this.context.datamap = DataHelper.data(System.getProperty("user.dir") + "//src//test//resources//testData/TestDataInput_Greenbox.xlsx",ctx.getCurrentXmlTest().getParameter("InputDataSheet"));
//datamap = DataHelper.data(System.getProperty("user.dir") + "//src//test//resources//testData/default.xlsx", "Sheet1");
String runHost=PropertiesFile.RunningHost;
this.mongoDB = new MongoDB();
this.mongoDB.connectMongoDB();
this.context.mongoDB = this.mongoDB;
this.context.STestName = ctx.getCurrentXmlTest().getName();
System.out.println("Executing Feature File: "+this.context.STestName);
System.out.println("Executing Feature file from : "+this.context.FeatureName);
if(runHost.equalsIgnoreCase("local")){
if(browser.equalsIgnoreCase("IE")){
DesiredCapabilities d = DesiredCapabilities.internetExplorer();
d.setCapability(InternetExplorerDriver.INTRODUCE_FLAKINESS_BY_IGNORING_SECURITY_DOMAINS,true);
d.setCapability("nativeEvents", false);
d.setCapability("INTRODUCE_FLAKINESS_BY_IGNORING_SECURITY_DOMAINS",true);
System.setProperty("webdriver.ie.driver", "CustomizedJar/IEDriverServer.exe");
WebDriver driver = new InternetExplorerDriver(d);
this.context.driver = driver;
this.context.driver.manage().window().maximize();
}
if(browser.equalsIgnoreCase("Firefox")){
System.setProperty("webdriver.firefox.profile", "default");
WebDriver driver = new FirefoxDriver();
this.context.driver = driver;
this.context.driver.manage().window().maximize();
}
if(browser.equalsIgnoreCase("Chrome")){
System.setProperty("webdriver.chrome.driver", "CustomizedJar/chromedriver.exe");
WebDriver driver = new ChromeDriver();
this.context.driver = driver;
this.context.driver.manage().window().maximize();
}
InetAddress IP=InetAddress.getLocalHost();
MachineIP=IP.getHostAddress();
//code to identify browser version
String browserVer =((JavascriptExecutor)this.context.driver).executeScript("return navigator.userAgent;").toString().toLowerCase();
System.out.println(browserVer.toLowerCase());
System.out.println(browser);
//String browser = "Chrome"; // We will pass this value from the TestNG i think , Specify the String (Mozilla / IE /Chrome)
String browsercase = browser.toLowerCase();
int start = browserVer.indexOf(browsercase)+browsercase.length()+1;
int End = start+4;
browserVersion = browserVer.substring(start, End).trim().replace(";", "");
System.out.println("Version:"+browserVer.substring(start, End).trim().replace(";", ""));
System.out.println("IP of local system is := "+MachineIP);
this.context.MachineIP =MachineIP;
this.context.browserVersion = browserVersion;
// this.driver.get(PropertiesFile.HubURL);
}
else{
DesiredCapabilities caps = new DesiredCapabilities();
//Browsers
if(browser.equalsIgnoreCase("IE")){
System.setProperty("webdriver.ie.driver", "CustomizedJar/IEDriverServer.exe");
caps = DesiredCapabilities.internetExplorer();
caps.setCapability(InternetExplorerDriver.INTRODUCE_FLAKINESS_BY_IGNORING_SECURITY_DOMAINS,true);
caps.setBrowserName("internet explorer");
caps.setCapability("nativeEvents", false);
caps.setCapability("INTRODUCE_FLAKINESS_BY_IGNORING_SECURITY_DOMAINS",true);
// caps.setCapability("");
}
if(browser.equalsIgnoreCase("Firefox")){
caps = DesiredCapabilities.firefox();
caps.setBrowserName("firefox");
}
if(browser.equalsIgnoreCase("Chrome")){
System.setProperty("webdriver.chrome.driver", "CustomizedJar/chromedriver.exe");
caps = DesiredCapabilities.chrome();
}
if(browser.equalsIgnoreCase("iPad"))
caps = DesiredCapabilities.ipad();
if(browser.equalsIgnoreCase("Android"))
caps = DesiredCapabilities.android();
//Platforms
if(platform.equalsIgnoreCase("Windows"))
caps.setPlatform(Platform.ANY);
if(platform.equalsIgnoreCase("XP"))
caps.setPlatform(Platform.XP);
if(platform.equalsIgnoreCase("MAC"))
caps.setPlatform(Platform.MAC);
if(platform.equalsIgnoreCase("Andorid"))
caps.setPlatform(Platform.ANDROID);
//Version
caps.setVersion(version);
RemoteWebDriver driver = new RemoteWebDriver(new URL(PropertiesFile.HubURL), caps);
this.context.driver = driver;
this.context.driver.manage().window().maximize();
caps.setCapability(CapabilityType.TAKES_SCREENSHOT, true);
Capabilities remotecap= ((RemoteWebDriver)this.context.driver).getCapabilities();
browserVersion = remotecap.getVersion();
MachineIP= NodeIPAddress.getIPOfNode((RemoteWebDriver) this.context.driver);
this.context.MachineIP = MachineIP;
this.context.browserVersion = browserVersion;
System.out.println("IP of Remote system is := "+MachineIP);
System.out.println("Version of Browser is := "+browserVersion);
}
this.context.driver.manage().deleteAllCookies();
//this.driver.get("http://www.google.com");
this.context.driver.manage().timeouts().implicitlyWait(20, TimeUnit.SECONDS);
//Configuration Ends Here
this.context.SRunAgainst = SRunAgainst;
this.strTestRunId = GenerateKey.GenerateExecutionId(this.context.STestName.replace(' ', '_'));
this.context.strTestRunId = this.strTestRunId;
this.mongoDB.startTestExecution(SuiteContext.SuiteRunId, strTestRunId, this.context.STestName , RunAgainst,browser, version);
this.mongoDB.updateTestExecution(this.strTestRunId, this.context.browserVersion, this.context.MachineIP);
}catch (Exception e){
System.out.println("Before Test: Exception Occured while executing feature :"+ctx.getCurrentXmlTest().getParameter(FeatureName));
e.printStackTrace();
}
}
/* ************************************* Cucumber Test Method ******************** */
/**
* Create one test method that will be invoked by TestNG and invoke the
* Cucumber runner within that method.
* @throws Throwable
*/
@Test(groups = "examples-testng", description = "Example of using TestNGCucumberRunner to invoke Cucumber")
public void runCukes(ITestContext istx) throws Throwable
{
System.out.println(Thread.currentThread().getId());
super.RunningOfCukes(OptionsSpecification(istx));
}
public String[] OptionsSpecification(ITestContext istx){
String[] Options_Runtime = new String[7];
String ProjectPath = PropertiesFile.GetProjectPath(RunCukesByCompositionTest.class.getProtectionDomain().getCodeSource().getLocation().getPath());
//Options_Runtime[0] = ProjectPath+"src/main/resources/com/adp/autopay/automation/features/"+istx.getCurrentXmlTest().getParameter("FeatureName");
Options_Runtime[0] = ProjectPath+"src/test/java/automation/features/"+istx.getCurrentXmlTest().getParameter("FeatureName");
Options_Runtime[1] = "--glue";
Options_Runtime[2] = "com/adp/autopay/automation/teststeps";
Options_Runtime[3] = "--glue";
Options_Runtime[4] = "com/adp/autopay/automation/cucumberRunner";
Options_Runtime[5] = "--tags";
Options_Runtime[6] = istx.getCurrentXmlTest().getParameter("Tags"); //@Ignore,@smoke
return Options_Runtime;
}
/* *********************************** After Test ***************************** */
@AfterTest
public void afterTest(ITestContext ctx) {
try {
System.out.println("After Test Method");
this.context = ObjectContainer.getInstance(ExecutionContext.class);
if (!(SuiteContext.SuiteName.equalsIgnoreCase("CommandLineExecutions"))) {
this.context.driver.quit();;
this.context.completeTestExecution();
this.context.disconnect();
ObjectContainer.removeClass(ExecutionContext.class);
File file = new File("C:/Temp/PerformanceSheet_" + ctx.getCurrentXmlTest().getName() + ".xls");
if (file.exists()) {
Date now = Calendar.getInstance().getTime();
DateFormat df = new SimpleDateFormat("yyyy_MM_dd_hh_mm_ss");
String theDate = df.format(now);
String FileName = "PerformanceSheet_" + ctx.getCurrentXmlTest().getName() + theDate + ".xls";
File file2 = new File("C:/Temp/" + FileName);
boolean success = file.renameTo(file2);
if (success) {
System.out.println("Performance Excel sheet generated: " + file2);
// try {
// File file_Remote = new File("//cdlnetappcon2/hwseshare/other/"+FileName);
// FileUtils.copyFile(file2,file_Remote);
// } catch (IOException e) {
// e.printStackTrace();
// }
}
}
} else {
ObjectContainer.removeClass(ExecutionContext.class);
}
}catch (Exception e){
ObjectContainer.removeClass(ExecutionContext.class);
}
}
/* *********************************** After Scenario ***************************** */
@AfterSuite
public void afterSuite(){
System.err.println("I am in After Suite Method");
MongoDB mongoDB = new MongoDB();
mongoDB.connectMongoDB();
mongoDB.endSuiteExecution(SuiteContext.SuiteRunId);
mongoDB.disconnectMongoDB();
if(mailList=="NoMailList" || mailList==null||mailList=="")
Mail.SendEmail(PropertiesFile.To, PropertiesFile.From, PropertiesFile.CC, SuiteContext.SuiteRunId);
else
Mail.SendEmail(mailList,PropertiesFile.From,PropertiesFile.CC,SuiteContext.SuiteRunId);
}
/* ************************************ Cucumber Configuration ********************************** */
}
<file_sep>package com.adp.autopay.automation.utility;
import com.adp.autopay.automation.cucumberRunner.RunCukesByCompositionTest;
import com.gargoylesoftware.htmlunit.BrowserVersion;
import org.openqa.selenium.htmlunit.HtmlUnitDriver;
import javax.mail.*;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeMessage;
import java.util.Properties;
import java.util.concurrent.TimeUnit;
public class Mail {
@SuppressWarnings("deprecation")
public static void SendEmail(String To,String From,String CC,String ExecutionSuiteID) {
Properties props = new Properties();
props.put("mail.smtp.host", "mailrelay.nj.adp.com");
props.put("mail.smtp.socketFactory.port", "465");
props.put("mail.smtp.socketFactory.class","javax.net.ssl.SSLSocketFactory");
props.put("mail.smtp.auth", "true");
props.put("mail.smtp.port", "25");
//System.err.println("http://cdlbenefitsqcre/AutomationWeb/Results?suiteId="+ExecutionSuiteID);
System.err.println( PropertiesFile.GetEnvironmentProperty("RESULTS_APP_SERVER_PATH") + "="+ExecutionSuiteID);
HtmlUnitDriver driver = new HtmlUnitDriver(BrowserVersion.INTERNET_EXPLORER_9);
// FirefoxDriver driver = new FirefoxDriver();
// driver.get("http://localhost:32323/Results?suiteId=SUITE_20140323_224454_395");
driver.manage().timeouts().implicitlyWait(1000, TimeUnit.SECONDS);
driver.manage().timeouts().pageLoadTimeout(1000, TimeUnit.SECONDS);
driver.get(PropertiesFile.GetEnvironmentProperty("RESULTS_APP_SERVER_PATH") + "="+ExecutionSuiteID);
String innerHtml1 = driver.getPageSource();
innerHtml1 += "<br><br><h2 style=\"color:#DC143C;\">\n\n\n\n\n\n\n\n Thanks,</h2>";
innerHtml1 += "<b style=\"color:#DC143C;\">Greenbox Automation Team</b><br>";
//innerHtml1 += "<br><br><b style=\"font-size:125%;color:Red;\">Please reach out Automation team with questions</b><br>";
//System.out.println(innerHtml1);
Session session = Session.getInstance(props,
new javax.mail.Authenticator() {
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication("username","password");
}
});
try {
Message message = new MimeMessage(session);
message.setFrom(new InternetAddress(From));
if(PropertiesFile.SendMail.equalsIgnoreCase("True")){
message.setRecipients(Message.RecipientType.TO,InternetAddress.parse(To));
if((PropertiesFile.CC != null)){
message.setRecipients(Message.RecipientType.CC,InternetAddress.parse(CC));
}
}
String SuiteName = RunCukesByCompositionTest.SuiteName;
if(SuiteName == null){
SuiteName = "Execution From Feature";
}
// String Environment = RunStory.Environment; +"("+Environment+")";
message.setSubject("Execution Summary: " + SuiteName);
//message.setContent(innerHtml1 + "\n\n please find the link for complete results " , "text/html");
message.setContent(innerHtml1, "text/html");
Transport.send(message);
System.err.println("Mail Done");
} catch (MessagingException e) {
throw new RuntimeException(e);
}
}
}
<file_sep>package com.adp.autopay.automation.commonlibrary;
import java.io.File;
import java.io.IOException;
import org.apache.commons.io.FileUtils;
import com.snowtide.PDF;
import com.snowtide.pdf.Document;
import com.snowtide.pdf.OutputTarget;
public class PDFOperations{
public static void DeleteFileifExist_PDForCSV(String ReportType,String FileName) throws IOException{
String Extension = null;
if(ReportType.equalsIgnoreCase("PDF")){
Extension = ".pdf";
}
if(ReportType.equalsIgnoreCase("EXCEL") || ReportType.equalsIgnoreCase("CSV")){
Extension = ".csv";
}
File file = new File("C:/Users/" + System.getProperty("user.name") + "/Downloads/"+FileName+Extension);
if(file.exists()){
file.delete();
System.out.println("*** previous file deleted");
return;
}
System.out.println("*** file doesnot existed before, you can save the file");
return;
}
public static boolean SavePDForCSVToStandardLocation(String FileName,String ReportType) throws IOException{
String Extension = null;
if(ReportType.equalsIgnoreCase("PDF")){
Extension = ".pdf";
}
if(ReportType.equalsIgnoreCase("EXCEL") || ReportType.equalsIgnoreCase("CSV")){
Extension = ".csv";
}
File file_src = new File("C:/Users/" + System.getProperty("user.name") + "/Downloads/"+FileName+Extension);
File file_dest = new File("C:/Temp/"+FileName+Extension);
if(file_src.exists()){
FileUtils.copyFile(file_src, file_dest);
return true;
}
return false;
}
public static boolean VerifyPDF(String PDFFileName , String Field) throws IOException{
String pdfFilePath = "C:/Temp/"+PDFFileName;
Document pdf = PDF.open(pdfFilePath);
StringBuilder text = new StringBuilder(1024);
pdf.pipe(new OutputTarget(text));
pdf.close();
String TextFromFile = text.toString();
if(TextFromFile.contains(Field)){
System.out.println("*** "+Field+" Found in the PDF");
return true;
}
else{
System.out.println("*** "+Field+" Not Found in the PDF");
return false;
}
}
}
<file_sep>package com.adp.autopay.automation.pagerepository;
import org.openqa.selenium.By;
public class CommonVan
{
}
| 105a33acb49136c77608123c1069016f2d9e0be2 | [
"Java"
] | 5 | Java | sudheergpalli/WebservicesCucumber | 5629229b28182590459e1a296ae2708dc380ceef | ee3da30f96787a8697a2276c245204d0a0f66715 | |
refs/heads/master | <repo_name>s6-debs/s6-portable-utils<file_sep>/src/skaembutils/s6-sort.c
/* ISC license. */
#include <string.h>
#include <strings.h>
#include <stdlib.h>
#include <errno.h>
#include <skalibs/allreadwrite.h>
#include <skalibs/sgetopt.h>
#include <skalibs/buffer.h>
#include <skalibs/strerr2.h>
#include <skalibs/stralloc.h>
#include <skalibs/genalloc.h>
#include <skalibs/djbunix.h>
#include <skalibs/skamisc.h>
#define USAGE "s6-sort [ -bcfru0 ]"
typedef int strncmp_t (char const *, char const *, size_t) ;
typedef strncmp_t *strncmp_t_ref ;
typedef int qsortcmp_t (void const *, void const *) ;
typedef qsortcmp_t *qsortcmp_t_ref ;
static int flagnoblanks = 0, flagreverse = 0, flaguniq = 0 ;
static strncmp_t_ref comp = &strncmp ;
static int compit (char const *s1, size_t n1, char const *s2, size_t n2)
{
int r ;
if (flagnoblanks)
{
while ((*s1 == ' ') || (*s1 == '\t')) (s1++, n1--) ;
while ((*s2 == ' ') || (*s2 == '\t')) (s2++, n2--) ;
}
r = (*comp)(s1, s2, n1 < n2 ? n1 : n2) ;
if (!r) r = n1 - n2 ;
return flagreverse ? -r : r ;
}
static int sacmp (stralloc const *a, stralloc const *b)
{
return compit(a->s, a->len - 1, b->s, b->len - 1) ;
}
static ssize_t slurplines (genalloc *lines, char sep)
{
ssize_t i = 0 ;
for (;; i++)
{
stralloc sa = STRALLOC_ZERO ;
int r = skagetln(buffer_0, &sa, sep) ;
if (!r) break ;
if ((r < 0) && ((errno != EPIPE) || !stralloc_catb(&sa, &sep, 1)))
return -1 ;
stralloc_shrink(&sa) ;
if (!genalloc_append(stralloc, lines, &sa)) return -1 ;
}
return i ;
}
static void uniq (genalloc *lines)
{
size_t len = genalloc_len(stralloc, lines) ;
stralloc *s = genalloc_s(stralloc, lines) ;
size_t i = 1 ;
for (; i < len ; i++)
if (!sacmp(s+i-1, s+i)) stralloc_free(s+i-1) ;
}
static ssize_t outputlines (stralloc const *s, size_t len)
{
size_t i = 0 ;
for (; i < len ; i++)
if (buffer_put(buffer_1, s[i].s, s[i].len) < 0) return 0 ;
return buffer_flush(buffer_1) ;
}
static int check (stralloc const *s, size_t len)
{
size_t i = 1 ;
for (; i < len ; i++)
if (sacmp(s+i-1, s+i) >= !flaguniq) return 0 ;
return 1 ;
}
int main (int argc, char const *const *argv)
{
genalloc lines = GENALLOC_ZERO ; /* array of stralloc */
char sep = '\n' ;
int flagcheck = 0 ;
PROG = "s6-sort" ;
{
subgetopt l = SUBGETOPT_ZERO ;
for (;;)
{
int opt = subgetopt_r(argc, argv, "bcfru0", &l) ;
if (opt == -1) break ;
switch (opt)
{
case 'b' : flagnoblanks = 1 ; break ;
case 'c' : flagcheck = 1 ; break ;
case 'f' : comp = &strncasecmp ; break ;
case 'r' : flagreverse = 1 ; break ;
case 'u' : flaguniq = 1 ; break ;
case '0' : sep = '\0' ; break ;
default : strerr_dieusage(100, USAGE) ;
}
}
argc -= l.ind ; argv += l.ind ;
}
if (slurplines(&lines, sep) < 0) strerr_diefu1sys(111, "read from stdin") ;
if (flagcheck) return !check(genalloc_s(stralloc, &lines), genalloc_len(stralloc, &lines)) ;
qsort(genalloc_s(stralloc, &lines), genalloc_len(stralloc, &lines), sizeof(stralloc), (qsortcmp_t_ref)&sacmp) ;
if (flaguniq) uniq(&lines) ;
if (!outputlines(genalloc_s(stralloc, &lines), genalloc_len(stralloc, &lines)))
strerr_diefu1sys(111, "write to stdout") ;
return 0 ;
}
<file_sep>/package/deps.mak
#
# This file has been generated by tools/gen-deps.sh
#
src/skaembutils/s6-basename.o src/skaembutils/s6-basename.lo: src/skaembutils/s6-basename.c
src/skaembutils/s6-cat.o src/skaembutils/s6-cat.lo: src/skaembutils/s6-cat.c
src/skaembutils/s6-chmod.o src/skaembutils/s6-chmod.lo: src/skaembutils/s6-chmod.c
src/skaembutils/s6-chown.o src/skaembutils/s6-chown.lo: src/skaembutils/s6-chown.c
src/skaembutils/s6-clock.o src/skaembutils/s6-clock.lo: src/skaembutils/s6-clock.c
src/skaembutils/s6-cut.o src/skaembutils/s6-cut.lo: src/skaembutils/s6-cut.c
src/skaembutils/s6-dirname.o src/skaembutils/s6-dirname.lo: src/skaembutils/s6-dirname.c
src/skaembutils/s6-dumpenv.o src/skaembutils/s6-dumpenv.lo: src/skaembutils/s6-dumpenv.c
src/skaembutils/s6-echo.o src/skaembutils/s6-echo.lo: src/skaembutils/s6-echo.c
src/skaembutils/s6-env.o src/skaembutils/s6-env.lo: src/skaembutils/s6-env.c src/include/s6-portable-utils/config.h
src/skaembutils/s6-expr.o src/skaembutils/s6-expr.lo: src/skaembutils/s6-expr.c
src/skaembutils/s6-false.o src/skaembutils/s6-false.lo: src/skaembutils/s6-false.c
src/skaembutils/s6-format-filter.o src/skaembutils/s6-format-filter.lo: src/skaembutils/s6-format-filter.c
src/skaembutils/s6-grep.o src/skaembutils/s6-grep.lo: src/skaembutils/s6-grep.c
src/skaembutils/s6-head.o src/skaembutils/s6-head.lo: src/skaembutils/s6-head.c
src/skaembutils/s6-hiercopy.o src/skaembutils/s6-hiercopy.lo: src/skaembutils/s6-hiercopy.c
src/skaembutils/s6-linkname.o src/skaembutils/s6-linkname.lo: src/skaembutils/s6-linkname.c
src/skaembutils/s6-ln.o src/skaembutils/s6-ln.lo: src/skaembutils/s6-ln.c
src/skaembutils/s6-ls.o src/skaembutils/s6-ls.lo: src/skaembutils/s6-ls.c
src/skaembutils/s6-maximumtime.o src/skaembutils/s6-maximumtime.lo: src/skaembutils/s6-maximumtime.c
src/skaembutils/s6-mkdir.o src/skaembutils/s6-mkdir.lo: src/skaembutils/s6-mkdir.c
src/skaembutils/s6-mkfifo.o src/skaembutils/s6-mkfifo.lo: src/skaembutils/s6-mkfifo.c
src/skaembutils/s6-nice.o src/skaembutils/s6-nice.lo: src/skaembutils/s6-nice.c
src/skaembutils/s6-nuke.o src/skaembutils/s6-nuke.lo: src/skaembutils/s6-nuke.c
src/skaembutils/s6-pause.o src/skaembutils/s6-pause.lo: src/skaembutils/s6-pause.c
src/skaembutils/s6-printenv.o src/skaembutils/s6-printenv.lo: src/skaembutils/s6-printenv.c
src/skaembutils/s6-quote-filter.o src/skaembutils/s6-quote-filter.lo: src/skaembutils/s6-quote-filter.c
src/skaembutils/s6-quote.o src/skaembutils/s6-quote.lo: src/skaembutils/s6-quote.c
src/skaembutils/s6-rename.o src/skaembutils/s6-rename.lo: src/skaembutils/s6-rename.c
src/skaembutils/s6-rmrf.o src/skaembutils/s6-rmrf.lo: src/skaembutils/s6-rmrf.c
src/skaembutils/s6-seq.o src/skaembutils/s6-seq.lo: src/skaembutils/s6-seq.c
src/skaembutils/s6-sleep.o src/skaembutils/s6-sleep.lo: src/skaembutils/s6-sleep.c
src/skaembutils/s6-sort.o src/skaembutils/s6-sort.lo: src/skaembutils/s6-sort.c
src/skaembutils/s6-sync.o src/skaembutils/s6-sync.lo: src/skaembutils/s6-sync.c
src/skaembutils/s6-tai64ndiff.o src/skaembutils/s6-tai64ndiff.lo: src/skaembutils/s6-tai64ndiff.c
src/skaembutils/s6-tail.o src/skaembutils/s6-tail.lo: src/skaembutils/s6-tail.c
src/skaembutils/s6-test.o src/skaembutils/s6-test.lo: src/skaembutils/s6-test.c
src/skaembutils/s6-touch.o src/skaembutils/s6-touch.lo: src/skaembutils/s6-touch.c
src/skaembutils/s6-true.o src/skaembutils/s6-true.lo: src/skaembutils/s6-true.c
src/skaembutils/s6-uniquename.o src/skaembutils/s6-uniquename.lo: src/skaembutils/s6-uniquename.c
src/skaembutils/s6-unquote-filter.o src/skaembutils/s6-unquote-filter.lo: src/skaembutils/s6-unquote-filter.c
src/skaembutils/s6-unquote.o src/skaembutils/s6-unquote.lo: src/skaembutils/s6-unquote.c
src/skaembutils/s6-update-symlinks.o src/skaembutils/s6-update-symlinks.lo: src/skaembutils/s6-update-symlinks.c
src/skaembutils/seekablepipe.o src/skaembutils/seekablepipe.lo: src/skaembutils/seekablepipe.c
s6-basename: EXTRA_LIBS := -lskarnet
s6-basename: src/skaembutils/s6-basename.o
s6-cat: EXTRA_LIBS := -lskarnet
s6-cat: src/skaembutils/s6-cat.o
s6-chmod: EXTRA_LIBS := -lskarnet
s6-chmod: src/skaembutils/s6-chmod.o
s6-chown: EXTRA_LIBS := -lskarnet
s6-chown: src/skaembutils/s6-chown.o
s6-clock: EXTRA_LIBS := -lskarnet ${SYSCLOCK_LIB}
s6-clock: src/skaembutils/s6-clock.o
s6-cut: EXTRA_LIBS := -lskarnet
s6-cut: src/skaembutils/s6-cut.o
s6-dirname: EXTRA_LIBS := -lskarnet
s6-dirname: src/skaembutils/s6-dirname.o
s6-dumpenv: EXTRA_LIBS := -lskarnet
s6-dumpenv: src/skaembutils/s6-dumpenv.o
s6-echo: EXTRA_LIBS := -lskarnet
s6-echo: src/skaembutils/s6-echo.o
s6-env: EXTRA_LIBS := -lskarnet
s6-env: src/skaembutils/s6-env.o
s6-expr: EXTRA_LIBS := -lskarnet
s6-expr: src/skaembutils/s6-expr.o
s6-false: EXTRA_LIBS :=
s6-false: src/skaembutils/s6-false.o
s6-format-filter: EXTRA_LIBS := -lskarnet
s6-format-filter: src/skaembutils/s6-format-filter.o
s6-grep: EXTRA_LIBS := -lskarnet
s6-grep: src/skaembutils/s6-grep.o
s6-head: EXTRA_LIBS := -lskarnet
s6-head: src/skaembutils/s6-head.o
s6-hiercopy: EXTRA_LIBS := -lskarnet
s6-hiercopy: src/skaembutils/s6-hiercopy.o
s6-linkname: EXTRA_LIBS := -lskarnet
s6-linkname: src/skaembutils/s6-linkname.o
s6-ln: EXTRA_LIBS := -lskarnet ${SOCKET_LIB} ${SYSCLOCK_LIB}
s6-ln: src/skaembutils/s6-ln.o
s6-ls: EXTRA_LIBS := -lskarnet
s6-ls: src/skaembutils/s6-ls.o
s6-maximumtime: EXTRA_LIBS := -lskarnet ${SYSCLOCK_LIB} ${SPAWN_LIB}
s6-maximumtime: src/skaembutils/s6-maximumtime.o
s6-mkdir: EXTRA_LIBS := -lskarnet
s6-mkdir: src/skaembutils/s6-mkdir.o
s6-mkfifo: EXTRA_LIBS := -lskarnet
s6-mkfifo: src/skaembutils/s6-mkfifo.o
s6-nice: EXTRA_LIBS := -lskarnet
s6-nice: src/skaembutils/s6-nice.o
s6-nuke: EXTRA_LIBS := -lskarnet
s6-nuke: src/skaembutils/s6-nuke.o
s6-pause: EXTRA_LIBS := -lskarnet
s6-pause: src/skaembutils/s6-pause.o
s6-printenv: EXTRA_LIBS := -lskarnet
s6-printenv: src/skaembutils/s6-printenv.o
s6-quote: EXTRA_LIBS := -lskarnet
s6-quote: src/skaembutils/s6-quote.o
s6-quote-filter: EXTRA_LIBS := -lskarnet
s6-quote-filter: src/skaembutils/s6-quote-filter.o
s6-rename: EXTRA_LIBS := -lskarnet
s6-rename: src/skaembutils/s6-rename.o
s6-rmrf: EXTRA_LIBS := -lskarnet
s6-rmrf: src/skaembutils/s6-rmrf.o
s6-seq: EXTRA_LIBS := -lskarnet
s6-seq: src/skaembutils/s6-seq.o
s6-sleep: EXTRA_LIBS := -lskarnet ${SYSCLOCK_LIB}
s6-sleep: src/skaembutils/s6-sleep.o
s6-sort: EXTRA_LIBS := -lskarnet
s6-sort: src/skaembutils/s6-sort.o
s6-sync: EXTRA_LIBS :=
s6-sync: src/skaembutils/s6-sync.o
s6-tai64ndiff: EXTRA_LIBS := -lskarnet
s6-tai64ndiff: src/skaembutils/s6-tai64ndiff.o
s6-tail: EXTRA_LIBS := -lskarnet
s6-tail: src/skaembutils/s6-tail.o
s6-test: EXTRA_LIBS := -lskarnet
s6-test: src/skaembutils/s6-test.o
s6-touch: EXTRA_LIBS := -lskarnet
s6-touch: src/skaembutils/s6-touch.o
s6-true: EXTRA_LIBS :=
s6-true: src/skaembutils/s6-true.o
s6-uniquename: EXTRA_LIBS := -lskarnet ${SOCKET_LIB} ${SYSCLOCK_LIB}
s6-uniquename: src/skaembutils/s6-uniquename.o
s6-unquote: EXTRA_LIBS := -lskarnet
s6-unquote: src/skaembutils/s6-unquote.o
s6-unquote-filter: EXTRA_LIBS := -lskarnet
s6-unquote-filter: src/skaembutils/s6-unquote-filter.o
s6-update-symlinks: EXTRA_LIBS := -lskarnet ${SOCKET_LIB} ${SYSCLOCK_LIB}
s6-update-symlinks: src/skaembutils/s6-update-symlinks.o
seekablepipe: EXTRA_LIBS := -lskarnet
seekablepipe: src/skaembutils/seekablepipe.o
<file_sep>/package/targets.mak
BIN_TARGETS := \
s6-basename \
s6-cat \
s6-chmod \
s6-chown \
s6-clock \
s6-cut \
s6-dirname \
s6-dumpenv \
s6-echo \
s6-env \
s6-expr \
s6-false \
s6-format-filter \
s6-grep \
s6-head \
s6-hiercopy \
s6-linkname \
s6-ln \
s6-ls \
s6-maximumtime \
s6-mkdir \
s6-mkfifo \
s6-nice \
s6-nuke \
s6-pause \
s6-printenv \
s6-quote \
s6-quote-filter \
s6-rename \
s6-rmrf \
s6-seq \
s6-sleep \
s6-sort \
s6-sync \
s6-tai64ndiff \
s6-tail \
s6-test \
s6-touch \
s6-true \
s6-uniquename \
s6-unquote \
s6-unquote-filter \
s6-update-symlinks \
seekablepipe
LIBEXEC_TARGETS :=
| c16f9b8b5cdc85ffbb81669d5995424f1a0a8d7f | [
"C",
"Makefile"
] | 3 | C | s6-debs/s6-portable-utils | 536eea15284be9a257b6f97f971e0d8d4a7e437b | f7d56d7f407e255548fb86d822ad1d4a1734de0c | |
refs/heads/master | <file_sep># RISC-V emulator in Go
Linux running at ~2Mhz:
```
OpenSBI v0.8
____ _____ ____ _____
/ __ \ / ____| _ \_ _|
| | | |_ __ ___ _ __ | (___ | |_) || |
| | | | '_ \ / _ \ '_ \ \___ \| _ < | |
| |__| | |_) | __/ | | |____) | |_) || |_
\____/| .__/ \___|_| |_|_____/|____/_____|
| |
|_|
Platform Name : riscv-virtio,qemu
Platform Features : timer,mfdeleg
Platform HART Count : 1
Boot HART ID : 0
Boot HART ISA : rv64imafdcbnsu
BOOT HART Features : pmp,scounteren,mcounteren,time
BOOT HART PMP Count : 16
Firmware Base : 0x80000000
Firmware Size : 96 KB
Runtime SBI Version : 0.2
MIDELEG : 0x0000000000000222
MEDELEG : 0x000000000000b109
PMP0 : 0x0000000080000000-0x000000008001ffff (A)
PMP1 : 0x0000000000000000-0xffffffffffffffff (A,R,W,X)
[ 0.000000] OF: fdt: Ignoring memory range 0x80000000 - 0x80200000
[ 0.000000] Linux version 5.4.0 (takahiro@takahiro-VirtualBox) (gcc version 10.1.0 (GCC)) #1 SMP Sun Oct 11 20:31:00 PDT 2020
[ 0.000000] initrd not found or empty - disabling initrd
[ 0.000000] Zone ranges:
[ 0.000000] DMA32 [mem 0x0000000080200000-0x0000000087ffffff]
[ 0.000000] Normal empty
[ 0.000000] Movable zone start for each node
[ 0.000000] Early memory node ranges
[ 0.000000] node 0: [mem 0x0000000080200000-0x0000000087ffffff]
[ 0.000000] Initmem setup node 0 [mem 0x0000000080200000-0x0000000087ffffff]
[ 0.000000] software IO TLB: mapped [mem 0x83e3c000-0x87e3c000] (64MB)
[ 0.000000] elf_hwcap is 0x112d
[ 0.000000] percpu: Embedded 17 pages/cpu s30680 r8192 d30760 u69632
[ 0.000000] Built 1 zonelists, mobility grouping on. Total pages: 31815
[ 0.000000] Kernel command line: root=/dev/vda rw ttyS0
[ 0.000000] Dentry cache hash table entries: 16384 (order: 5, 131072 bytes, linear)
[ 0.000000] Inode-cache hash table entries: 8192 (order: 4, 65536 bytes, linear)
[ 0.000000] Sorting __ex_table...
[ 0.000000] mem auto-init: stack:off, heap alloc:off, heap free:off
[ 0.000000] Memory: 51976K/129024K available (6166K kernel code, 387K rwdata, 1962K rodata, 213K init, 305K bss, 77048K reserved, 0K cma-reserved)
[ 0.000000] SLUB: HWalign=64, Order=0-3, MinObjects=0, CPUs=1, Nodes=1
[ 0.000000] rcu: Hierarchical RCU implementation.
[ 0.000000] rcu: RCU restricting CPUs from NR_CPUS=8 to nr_cpu_ids=1.
[ 0.000000] rcu: RCU calculated value of scheduler-enlistment delay is 25 jiffies.
[ 0.000000] rcu: Adjusting geometry for rcu_fanout_leaf=16, nr_cpu_ids=1
[ 0.000000] NR_IRQS: 0, nr_irqs: 0, preallocated irqs: 0
[ 0.000000] plic: mapped 53 interrupts with 1 handlers for 2 contexts.
[ 0.000000] riscv_timer_init_dt: Registering clocksource cpuid [0] hartid [0]
[ 0.000000] clocksource: riscv_clocksource: mask: 0xffffffffffffffff max_cycles: 0x24e6a1710, max_idle_ns: 440795202120 ns
[ 0.000330] sched_clock: 64 bits at 10MHz, resolution 100ns, wraps every 4398046511100ns
[ 0.006350] Console: colour dummy device 80x25
[ 0.061158] printk: console [tty0] enabled
[ 0.064907] Calibrating delay loop (skipped), value calculated using timer frequency.. 20.00 BogoMIPS (lpj=40000)
[ 0.071021] pid_max: default: 32768 minimum: 301
[ 0.080459] Mount-cache hash table entries: 512 (order: 0, 4096 bytes, linear)
[ 0.086266] Mountpoint-cache hash table entries: 512 (order: 0, 4096 bytes, linear)
[ 0.147251] rcu: Hierarchical SRCU implementation.
[ 0.159109] smp: Bringing up secondary CPUs ...
[ 0.162698] smp: Brought up 1 node, 1 CPU
[ 0.176101] devtmpfs: initialized
[ 0.214369] random: get_random_u32 called from bucket_table_alloc.isra.0+0x4e/0x154 with crng_init=0
[ 0.229596] clocksource: jiffies: mask: 0xffffffff max_cycles: 0xffffffff, max_idle_ns: 7645041785100000 ns
[ 0.235644] futex hash table entries: 256 (order: 2, 16384 bytes, linear)
[ 0.252619] NET: Registered protocol family 16
[ 1.004435] vgaarb: loaded
[ 1.023176] SCSI subsystem initialized
[ 1.040987] usbcore: registered new interface driver usbfs
[ 1.046258] usbcore: registered new interface driver hub
[ 1.051057] usbcore: registered new device driver usb
[ 1.085403] clocksource: Switched to clocksource riscv_clocksource
[ 1.368901] NET: Registered protocol family 2
[ 1.401444] tcp_listen_portaddr_hash hash table entries: 256 (order: 0, 4096 bytes, linear)
[ 1.407649] TCP established hash table entries: 1024 (order: 1, 8192 bytes, linear)
[ 1.414561] TCP bind hash table entries: 1024 (order: 2, 16384 bytes, linear)
[ 1.419342] TCP: Hash tables configured (established 1024 bind 1024)
[ 1.425572] UDP hash table entries: 256 (order: 1, 8192 bytes, linear)
[ 1.430003] UDP-Lite hash table entries: 256 (order: 1, 8192 bytes, linear)
[ 1.438657] NET: Registered protocol family 1
[ 1.457454] RPC: Registered named UNIX socket transport module.
[ 1.460850] RPC: Registered udp transport module.
[ 1.463561] RPC: Registered tcp transport module.
[ 1.466885] RPC: Registered tcp NFSv4.1 backchannel transport module.
[ 1.470375] PCI: CLS 0 bytes, default 64
[ 1.503201] workingset: timestamp_bits=62 max_order=14 bucket_order=0
[ 2.030752] NFS: Registering the id_resolver key type
[ 2.034478] Key type id_resolver registered
[ 2.037728] Key type id_legacy registered
[ 2.041286] nfs4filelayout_init: NFSv4 File Layout Driver Registering...
[ 2.049276] 9p: Installing v9fs 9p2000 file system support
[ 2.068910] NET: Registered protocol family 38
[ 2.073114] Block layer SCSI generic (bsg) driver version 0.4 loaded (major 253)
[ 2.078607] io scheduler mq-deadline registered
[ 2.081881] io scheduler kyber registered
[ 3.816839] Serial: 8250/16550 driver, 4 ports, IRQ sharing disabled
[ 3.869830] 10000000.uart: ttyS0 at MMIO 0x10000000 (irq = 10, base_baud = 230400) is a 16550A
[ 8.348990] printk: console [ttyS0] enabled
[ 8.431798] [drm] radeon kernel modesetting enabled.
[ 8.724696] loop: module loaded
[ 8.883840] virtio_blk virtio0: [vda] 204800 512-byte logical blocks (105 MB/100 MiB)
[ 9.001852] libphy: Fixed MDIO Bus: probed
[ 9.089842] e1000e: Intel(R) PRO/1000 Network Driver - 3.2.6-k
[ 9.149054] e1000e: Copyright(c) 1999 - 2015 Intel Corporation.
[ 9.213276] ehci_hcd: USB 2.0 'Enhanced' Host Controller (EHCI) Driver
[ 9.272730] ehci-pci: EHCI PCI platform driver
[ 9.333478] ehci-platform: EHCI generic platform driver
[ 9.394449] ohci_hcd: USB 1.1 'Open' Host Controller (OHCI) Driver
[ 9.453573] ohci-pci: OHCI PCI platform driver
[ 9.514010] ohci-platform: OHCI generic platform driver
[ 9.581489] usbcore: registered new interface driver uas
[ 9.642718] usbcore: registered new interface driver usb-storage
[ 9.708040] mousedev: PS/2 mouse device common for all mice
[ 9.786904] usbcore: registered new interface driver usbhid
[ 9.846033] usbhid: USB HID core driver
[ 9.951406] NET: Registered protocol family 10
[ 10.039863] Segment Routing with IPv6
[ 10.102449] sit: IPv6, IPv4 and MPLS over IPv4 tunneling driver
[ 10.189783] NET: Registered protocol family 17
[ 10.260784] 9pnet: Installing 9P2000 support
[ 10.322578] Key type dns_resolver registered
[ 10.423380] EXT4-fs (vda): mounting ext2 file system using the ext4 subsystem
[ 10.536168] EXT4-fs (vda): warning: mounting unchecked fs, running e2fsck is recommended
[ 10.613433] EXT4-fs (vda): mounted filesystem without journal. Opts: (null)
[ 10.673739] VFS: Mounted root (ext2 filesystem) on device 254:0.
[ 10.737193] devtmpfs: mounted
[ 10.802606] Freeing unused kernel memory: 212K
[ 10.861531] This architecture does not have kernel memory protection.
[ 10.920852] Run /sbin/init as init process
```<file_sep>module rvgo
go 1.16
require (
github.com/gdamore/tcell/v2 v2.4.0 // indirect
github.com/stretchr/testify v1.7.0
)
<file_sep>package main
import (
"fmt"
"io/ioutil"
"os"
"path/filepath"
"strings"
"testing"
"time"
"github.com/stretchr/testify/assert"
)
func TestRiscvTests(t *testing.T) {
files, err := ioutil.ReadDir("testdata")
assert.NoError(t, err)
for _, file := range files {
if strings.HasSuffix(file.Name(), ".dump") {
continue
}
// if file.Name() != "rv64uf-p-fcvt_w" {
// continue
// }
// var err error
// debugFile, err = os.Create("trace.txt")
// assert.NoError(t, err)
// defer debugFile.Close()
// DEBUG = true
t.Run(file.Name(), func(t *testing.T) {
mem := make([]byte, 0x10000)
entry, err := loadElf(filepath.Join("testdata", file.Name()), mem)
assert.NoError(t, err)
cpu := NewCPU(mem, entry, nil, nil)
for {
cpu.step()
exitcode := mem[0x1000]
if exitcode != 0 {
assert.Equal(t, uint8(1), exitcode, "failing exitcode %d recieved", exitcode)
return
}
}
})
}
}
func TestLinux(t *testing.T) {
var err error
debugFile, err = os.Create("trace.txt")
assert.NoError(t, err)
defer debugFile.Close()
DEBUG = false
mem := make([]byte, 0x10000000)
entry, err := loadElf(filepath.Join("linux", "fw_payload.elf"), mem)
assert.NoError(t, err)
rootfs, err := ioutil.ReadFile(filepath.Join("linux", "rootfs.img"))
assert.NoError(t, err)
cpu := NewCPU(mem, entry, rootfs, nil)
start := time.Now()
defer func() {
end := time.Now()
err := recover()
fmt.Printf("finished at cycle: %d -- pc==%x\n", cpu.count, cpu.pc)
fmt.Printf("failed with: %v\n", err)
fmt.Printf("%0.4f MHz\n", float64(cpu.count*1000)/float64(end.UnixNano()-start.UnixNano()))
assert.GreaterOrEqual(t, cpu.count, uint64(128439363))
}()
for {
cpu.step()
if cpu.wfi {
return
}
}
}
<file_sep>package main
import (
"bytes"
"debug/elf"
_ "embed"
"fmt"
"io/ioutil"
"math"
"os"
"path/filepath"
"strings"
"unicode/utf8"
"unsafe"
"github.com/gdamore/tcell/v2"
)
var MEMORYBASE uint64 = 0x80000000
var DEBUG = false
var debugFile *os.File
const (
FFLAGS = 0x001
FRM = 0x002
FCSR = 0x003
MVENDORID = 0xF11
MARCHID = 0xF12
MIMPID = 0xF13
MHARTID = 0xF14
MSTATUS = 0x300
MISA = 0x301
MEDELEG = 0x302
MIDELEG = 0x303
MIE = 0x304
MTVEC = 0x305
MCOUNTEREN = 0x306
MSCRATCH = 0x340
MEPC = 0x341
MCAUSE = 0x342
MTVAL = 0x343
MIP = 0x344
SSTATUS = 0x100
SEDELEG = 0x102
SIDELEG = 0x103
SIE = 0x104
STVEC = 0x105
SCOUNTEREN = 0x106
SSCRATCH = 0x140
SEPC = 0x141
SCAUSE = 0x142
STVAL = 0x143
SIP = 0x144
SATP = 0x180
USTATUS = 0x000
UIE = 0x004
UTVEC = 0x005
USCRATCH = 0x040
UEPC = 0x041
UCAUSE = 0x042
UTVAL = 0x043
UIP = 0x044
CYCLE = 0xC00
TIME = 0xC01
INSTRET = 0xC02
)
const (
MIP_MEIP = 0x800
MIP_MTIP = 0x080
MIP_MSIP = 0x008
MIP_SEIP = 0x200
MIP_STIP = 0x020
MIP_SSIP = 0x002
)
type Privilege uint8
const (
User Privilege = 0
Supervisor Privilege = 1
Hypervisor Privilege = 2
Machine Privilege = 3
)
type AddressMode uint8
const (
None AddressMode = 0
SV39 AddressMode = 8
SV48 AddressMode = 9
)
type Access uint8
const (
Read Access = 0
Write Access = 1
Execute Access = 2
Unknown Access = 3
)
//go:embed dtb/dtb.dtb
var dtb []byte
type CPU struct {
pc uint64
mem []byte
mem32 []uint32
mem64 []uint64
x [32]int64
f [32]float64
csr [4096]uint64
priv Privilege
mode AddressMode
wfi bool
reservation uint64
reservationSet bool
uart *Uart
plic Plic
clint Clint
disk VirtioBlock
count uint64
}
func NewCPU(mem []byte, pc uint64, disk []byte, screen tcell.Screen) CPU {
cpu := CPU{
pc: pc,
mem: mem,
mem32: *((*[]uint32)(unsafe.Pointer(&mem))),
mem64: *((*[]uint64)(unsafe.Pointer(&mem))),
priv: Machine,
uart: NewUart(screen),
plic: NewPlic(),
clint: NewClint(),
}
cpu.disk = NewVirtioBlock(disk, &cpu)
// TODO: Why?
cpu.x[0xb] = 0x1020
return cpu
}
func (cpu *CPU) run() {
for {
cpu.step()
}
}
func (cpu *CPU) step() {
addr := cpu.pc
ok, trap := cpu.stepInner()
if !ok {
cpu.exception(trap, addr)
}
cpu.count++
cpu.disk.step()
cpu.uart.step(cpu.count)
cpu.clint.step(cpu.count, &cpu.csr[MIP])
cpu.plic.step(cpu.count, cpu.uart.interrupting, cpu.disk.interruptStatus&0b1 == 1, &cpu.csr[MIP])
cpu.interrupt(cpu.pc)
cpu.csr[CYCLE] = cpu.count * 8
}
func (cpu *CPU) stepInner() (bool, Trap) {
if cpu.wfi {
if cpu.readcsr(MIE)&cpu.readcsr(MIP) != 0 {
cpu.wfi = false
}
return true, Trap{}
}
instr, ok, trap := cpu.fetch()
if !ok {
cpu.pc += 4 // TODO: okay?
return false, trap
}
addr := cpu.pc
if DEBUG {
// if cpu.count > 10084750 {
// if cpu.count%100000 == 0 {
var regs []string
for _, r := range cpu.x {
regs = append(regs, fmt.Sprintf("%x", uint64(r)))
}
// memHash := sha1.Sum(cpu.mem)
// csrSlice := cpu.csr[:]
// csrBytes := *((*[]uint8)(unsafe.Pointer(&csrSlice)))
// csrHash := sha1.Sum(csrBytes)
// fmt.Fprintf(debugFile, "%08d -- [%08x]: %08x [%s] mem=%0x csrs=%0x\n", cpu.count, cpu.pc, instr, strings.Join(regs, ", "), memHash, csrHash)
fmt.Fprintf(debugFile, "%08d -- [%08x]: %08x [%s]\n", cpu.count, cpu.pc, instr, strings.Join(regs, ", "))
// }
}
if instr&0b11 == 0b11 {
cpu.pc += 4
} else {
cpu.pc += 2
instr = cpu.decompress(instr & 0xFFFF)
}
ok, trap = cpu.exec(instr, addr)
cpu.x[0] = 0
if !ok {
return false, trap
}
return true, Trap{}
}
func (cpu *CPU) fetch() (uint32, bool, Trap) {
v := uint32(0)
if cpu.pc&0xfff <= 0x1000-4 { // instruction is within a single page
paddr, ok := cpu.virtualToPhysical(cpu.pc, Execute)
if !ok {
return 0, false, Trap{reason: InstructionPageFault, value: cpu.pc}
}
if paddr >= MEMORYBASE && paddr%4 == 0 {
memaddr := paddr - MEMORYBASE
v = uint32(cpu.mem32[memaddr/4])
} else {
for i := uint64(0); i < 4; i++ {
x := cpu.readphysical(paddr + i)
v |= uint32(x) << (i * 8)
}
}
} else { // instruction is across two pages
for i := uint64(0); i < 4; i++ {
paddr, ok := cpu.virtualToPhysical(cpu.pc+i, Execute)
if !ok {
return 0, false, Trap{reason: InstructionPageFault, value: cpu.pc + i}
}
x := cpu.readphysical(paddr)
v |= uint32(x) << (i * 8)
}
}
return v, true, Trap{}
}
type TrapReason uint64
const (
UserSoftwareInterrupt TrapReason = 0x8000000000000000
SupervisorSoftwareInterrupt TrapReason = 0x8000000000000001
HypervisorSoftwareInterrupt TrapReason = 0x8000000000000002
MachineSoftwareInterrupt TrapReason = 0x8000000000000003
UserTimerInterrupt TrapReason = 0x8000000000000004
SupervisorTimerInterrupt TrapReason = 0x8000000000000005
HypervisorTimerInterrupt TrapReason = 0x8000000000000006
MachineTimerInterrupt TrapReason = 0x8000000000000007
UserExternalInterrupt TrapReason = 0x8000000000000008
SupervisorExternalInterrupt TrapReason = 0x8000000000000009
HypervisorExternalInterrupt TrapReason = 0x800000000000000A
MachineExternalInterrupt TrapReason = 0x800000000000000B
InstructionAddressMisaligned TrapReason = 0x0000000000000000
InstructionAccessFault TrapReason = 0x0000000000000001
IllegalInstruction TrapReason = 0x0000000000000002
Breakpoint TrapReason = 0x0000000000000003
LoadAddressMisaligned TrapReason = 0x0000000000000004
LoadAccessFault TrapReason = 0x0000000000000005
StoreAddressMisaligned TrapReason = 0x0000000000000006
StoreAccessFault TrapReason = 0x0000000000000007
EnvironmentCallFromUMode TrapReason = 0x0000000000000008
EnvironmentCallFromSMode TrapReason = 0x0000000000000009
EnvironmentCallFromHMode TrapReason = 0x000000000000000A
EnvironmentCallFromMMode TrapReason = 0x000000000000000B
InstructionPageFault TrapReason = 0x000000000000000C
LoadPageFault TrapReason = 0x000000000000000D
StorePageFault TrapReason = 0x000000000000000F
)
type Trap struct {
reason TrapReason
value uint64
}
func (cpu *CPU) exception(trap Trap, instructionaddr uint64) {
cpu.trap(trap.reason, trap.value, instructionaddr, false)
}
func (cpu *CPU) interrupt(pc uint64) {
minterrupt := cpu.readcsr(MIP) & cpu.readcsr(MIE)
if minterrupt&MIP_MEIP != 0 {
panic("nyi - handle MachineExternalInterrupt")
}
if minterrupt&MIP_MSIP != 0 {
panic("nyi - handle MachineSoftwareInterrupt")
}
if minterrupt&MIP_MTIP != 0 {
traptaken := cpu.trap(MachineTimerInterrupt, cpu.pc, pc, true)
if traptaken {
cpu.writecsr(MIP, cpu.readcsr(MIP)&^MIP_MTIP)
cpu.wfi = false
}
}
if minterrupt&MIP_SEIP != 0 {
traptaken := cpu.trap(SupervisorExternalInterrupt, cpu.pc, pc, true)
if traptaken {
cpu.writecsr(MIP, cpu.readcsr(MIP)&^MIP_SEIP)
cpu.wfi = false
}
}
if minterrupt&MIP_SSIP != 0 {
traptaken := cpu.trap(SupervisorSoftwareInterrupt, cpu.pc, pc, true)
if traptaken {
cpu.writecsr(MIP, cpu.readcsr(MIP)&^MIP_SSIP)
cpu.wfi = false
}
}
if minterrupt&MIP_STIP != 0 {
traptaken := cpu.trap(SupervisorTimerInterrupt, cpu.pc, pc, true)
if traptaken {
cpu.writecsr(MIP, cpu.readcsr(MIP)&^MIP_STIP)
cpu.wfi = false
}
}
}
func (cpu *CPU) trap(reason TrapReason, value, addr uint64, isInterrupt bool) bool {
// if reason != 0x8000000000000007 && reason != 0x8000000000000005 {
// if reason == 0x8000000000000009 {
// fmt.Fprintf(debugFile, "Handling trap: %d, %08x, %08x, %08x\n", cpu.count, reason, value, addr)
// }
var mdeleg, sdeleg uint64
if isInterrupt {
mdeleg, sdeleg = cpu.readcsr(MIDELEG), cpu.readcsr(SIDELEG)
} else {
mdeleg, sdeleg = cpu.readcsr(MEDELEG), cpu.readcsr(SEDELEG)
}
pos := uint64(reason) & 0xFFFF
fromPriv := cpu.priv
var handlePriv Privilege
var status, ie uint64
var epcAddr, causeAddr, tvalAddr, tvecAddr uint16
if (mdeleg>>pos)&1 == 0 {
handlePriv = Machine
status = cpu.readcsr(MSTATUS)
ie = cpu.readcsr(MIE)
epcAddr, causeAddr, tvalAddr, tvecAddr = MEPC, MCAUSE, MTVAL, MTVEC
} else if (sdeleg>>pos)&1 == 0 {
handlePriv = Supervisor
status = cpu.readcsr(SSTATUS)
ie = cpu.readcsr(SIE)
epcAddr, causeAddr, tvalAddr, tvecAddr = SEPC, SCAUSE, STVAL, STVEC
} else {
handlePriv = User
status = cpu.readcsr(USTATUS)
ie = cpu.readcsr(UIE)
epcAddr, causeAddr, tvalAddr, tvecAddr = UEPC, UCAUSE, UTVAL, UTVEC
}
if isInterrupt {
if handlePriv < fromPriv {
return false
} else if handlePriv == fromPriv {
switch fromPriv {
case Machine:
if (status>>3)&1 == 0 {
return false
}
case Supervisor:
if (status>>1)&1 == 0 {
return false
}
case User:
if (status>>0)&1 == 0 {
return false
}
default:
panic("invalid privilege")
}
}
switch reason {
case UserSoftwareInterrupt:
if (ie>>0)&1 == 0 {
return false
}
case SupervisorSoftwareInterrupt:
if (ie>>1)&1 == 0 {
return false
}
case MachineSoftwareInterrupt:
if (ie>>3)&1 == 0 {
return false
}
case UserTimerInterrupt:
if (ie>>4)&1 == 0 {
return false
}
case SupervisorTimerInterrupt:
if (ie>>5)&1 == 0 {
return false
}
case MachineTimerInterrupt:
if (ie>>7)&1 == 0 {
return false
}
case UserExternalInterrupt:
if (ie>>8)&1 == 0 {
return false
}
case SupervisorExternalInterrupt:
if (ie>>9)&1 == 0 {
return false
}
case MachineExternalInterrupt:
if (ie>>11)&1 == 0 {
return false
}
}
}
cpu.priv = handlePriv
cpu.writecsr(epcAddr, addr)
cpu.writecsr(causeAddr, uint64(reason))
cpu.writecsr(tvalAddr, value)
cpu.pc = cpu.readcsr(tvecAddr)
if (cpu.pc & 0b11) != 0 {
panic("vector type address")
cpu.pc = (cpu.pc>>2)<<2 + (4 * (uint64(reason) & 0xFFFF))
}
switch cpu.priv {
case Machine:
status := cpu.readcsr(MSTATUS)
mie := (status >> 3) & 0b1
status = (status &^ 0x1888) | mie<<7 | uint64(fromPriv)<<11
cpu.writecsr(MSTATUS, status)
case Supervisor:
status := cpu.readcsr(SSTATUS)
sie := (status >> 1) & 0b1
status = (status &^ 0x122) | sie<<5 | uint64(fromPriv&1)<<8
cpu.writecsr(SSTATUS, status)
case User:
panic("nyi - user mode exception handler")
default:
panic("invalid privilege")
}
return true
}
type I struct {
imm int32
rs1 uint32
funct3 uint32
rd uint32
}
func parseI(instr uint32) I {
imm := uint32(0)
if (instr>>31)&0b1 == 0b1 {
imm = 0xfffff800
}
return I{
imm: int32(imm | (instr>>20)&0x000007ff),
rs1: (instr >> 15) & 0b11111,
funct3: (instr >> 12) & 0b111,
rd: (instr >> 7) & 0b11111,
}
}
type S struct {
imm int32
rs1 uint32
funct3 uint32
rs2 uint32
}
func parseS(instr uint32) S {
imm := uint32(0)
if (instr>>31)&0b1 == 0b1 {
imm = 0xfffff800
}
return S{
imm: int32(imm | ((instr>>25)&0x3f)<<5 | (instr>>7)&0x1f), // 1 + 6 + 5
rs1: (instr >> 15) & 0b11111, // 5
rs2: (instr >> 20) & 0b11111, // 5
funct3: (instr >> 12) & 0b111, // 3
}
}
type B struct {
imm int32
rs1 uint32
funct3 uint32
rs2 uint32
}
func parseB(instr uint32) B {
imm := uint32(0)
if (instr>>31)&0b1 == 0b1 {
imm = 0xfffff000
}
return B{
imm: int32(imm | ((instr>>25)&0x3f)<<5 | (instr>>7)&0x1e | (instr>>7)&0b1<<11), // 1 + 6 + 4 + 1
rs1: (instr >> 15) & 0b11111, // 5
rs2: (instr >> 20) & 0b11111, // 5
funct3: (instr >> 12) & 0b111, // 3
}
}
type U struct {
imm int64
rd uint32
}
func parseU(instr uint32) U {
imm := uint64(0)
if (instr>>31)&0b1 == 0b1 {
imm = 0xffffffff00000000
}
return U{
imm: int64(imm | uint64(instr)&0xfffff000),
rd: (instr >> 7) & 0b11111,
}
}
type J struct {
imm int32
rd uint32
}
func parseJ(instr uint32) J {
imm := uint32(0)
if (instr>>31)&0b1 == 0b1 {
imm = 0xfff00000
}
return J{
imm: int32(imm | (instr & 0x000ff000) | (instr&0x00100000)>>9 | (instr&0x7fe00000)>>20),
rd: (instr >> 7) & 0b11111,
}
}
type CSR struct {
csr uint32
rs uint32
funct3 uint32
rd uint32
}
func parseCSR(instr uint32) CSR {
return CSR{
csr: (instr >> 20) & 0x00000fff,
rs: (instr >> 15) & 0b11111,
funct3: (instr >> 12) & 0b111,
rd: (instr >> 7) & 0b11111,
}
}
type R struct {
funct7 uint32
rs2 uint32
rs1 uint32
funct3 uint32
rd uint32
}
func parseR(instr uint32) R {
return R{
funct7: (instr >> 25) & 0b1111111,
rs2: (instr >> 20) & 0b11111,
rs1: (instr >> 15) & 0b11111,
funct3: (instr >> 12) & 0b111,
rd: (instr >> 7) & 0b11111,
}
}
func (cpu *CPU) exec(instr uint32, addr uint64) (bool, Trap) {
switch instr & 0x7f {
case 0b0110111: // LUI
op := parseU(instr)
cpu.x[op.rd] = int64(op.imm)
case 0b0010111: // AUIPC
op := parseU(instr)
cpu.x[op.rd] = int64(addr) + op.imm
case 0b1101111: // JAL
op := parseJ(instr)
cpu.x[op.rd] = int64(cpu.pc)
cpu.pc = addr + uint64(int64(op.imm))
case 0b1100111: // JALR
op := parseI(instr)
rd := op.rd
// TODO: Check on this?
// if rd == 0 {
// rd = 1
// }
t := int64(cpu.pc)
cpu.pc = (uint64(cpu.x[op.rs1]+int64(op.imm)) >> 1) << 1
cpu.x[rd] = t
case 0b1100011:
op := parseB(instr)
switch op.funct3 {
case 0b000: // BEQ
if cpu.x[op.rs1] == cpu.x[op.rs2] {
cpu.pc = addr + uint64(op.imm)
}
case 0b001: // BNE
if cpu.x[op.rs1] != cpu.x[op.rs2] {
cpu.pc = addr + uint64(op.imm)
}
case 0b100: // BLT
if cpu.x[op.rs1] < cpu.x[op.rs2] {
cpu.pc = addr + uint64(op.imm)
}
case 0b101: // BGE
if cpu.x[op.rs1] >= cpu.x[op.rs2] {
cpu.pc = addr + uint64(op.imm)
}
case 0b110: // BLTU
if uint64(cpu.x[op.rs1]) < uint64(cpu.x[op.rs2]) {
cpu.pc = addr + uint64(op.imm)
}
case 0b111: // BGEU
if uint64(cpu.x[op.rs1]) >= uint64(cpu.x[op.rs2]) {
cpu.pc = addr + uint64(op.imm)
}
default:
panic(fmt.Sprintf("nyi - invalid instruction %x", instr))
}
case 0b0000011:
op := parseI(instr)
switch op.funct3 {
case 0b000: // LB
data, ok, trap := cpu.readuint8(uint64(cpu.x[op.rs1] + int64(op.imm)))
if !ok {
return false, trap
}
cpu.x[op.rd] = int64(int8(data))
case 0b001: // LH
data, ok, trap := cpu.readuint16(uint64(cpu.x[op.rs1] + int64(op.imm)))
if !ok {
return false, trap
}
cpu.x[op.rd] = int64(int16(data))
case 0b010: // LW
data, ok, trap := cpu.readuint32(uint64(cpu.x[op.rs1] + int64(op.imm)))
if !ok {
return false, trap
}
cpu.x[op.rd] = int64(int32(data))
case 0b100: // LBU
data, ok, trap := cpu.readuint8(uint64(cpu.x[op.rs1] + int64(op.imm)))
if !ok {
return false, trap
}
cpu.x[op.rd] = int64(data)
case 0b101: // LHU
data, ok, trap := cpu.readuint16(uint64(cpu.x[op.rs1] + int64(op.imm)))
if !ok {
return false, trap
}
cpu.x[op.rd] = int64(data)
case 0b011: // LD
data, ok, trap := cpu.readuint64(uint64(cpu.x[op.rs1] + int64(op.imm)))
if !ok {
return false, trap
}
cpu.x[op.rd] = int64(data)
case 0b110: // LWU
data, ok, trap := cpu.readuint32(uint64(cpu.x[op.rs1] + int64(op.imm)))
if !ok {
return false, trap
}
cpu.x[op.rd] = int64(uint64(data))
default:
panic(fmt.Sprintf("nyi - invalid instruction %x", instr))
}
case 0b0100011:
op := parseS(instr)
switch op.funct3 {
case 0b000: // SB
ok, trap := cpu.writeuint8(uint64(cpu.x[op.rs1]+int64(op.imm)), uint8(cpu.x[op.rs2]))
if !ok {
return false, trap
}
case 0b001: // SH
ok, trap := cpu.writeuint16(uint64(cpu.x[op.rs1]+int64(op.imm)), uint16(cpu.x[op.rs2]))
if !ok {
return false, trap
}
case 0b010: // SW
ok, trap := cpu.writeuint32(uint64(cpu.x[op.rs1]+int64(op.imm)), uint32(cpu.x[op.rs2]))
if !ok {
return false, trap
}
case 0b011: // SD
ok, trap := cpu.writeuint64(uint64(cpu.x[op.rs1]+int64(op.imm)), uint64(cpu.x[op.rs2]))
if !ok {
return false, trap
}
default:
panic(fmt.Sprintf("nyi - invalid instruction %x", instr))
}
case 0b0010011:
op := parseI(instr)
switch op.funct3 {
case 0b000: // ADDI
cpu.x[op.rd] = cpu.x[op.rs1] + int64(op.imm)
case 0b010: // SLTI
if cpu.x[op.rs1] < int64(op.imm) {
cpu.x[op.rd] = 1
} else {
cpu.x[op.rd] = 0
}
case 0b011: // SLTIU
if uint64(cpu.x[op.rs1]) < uint64(int64(op.imm)) {
cpu.x[op.rd] = 1
} else {
cpu.x[op.rd] = 0
}
case 0b100: // XORI
cpu.x[op.rd] = cpu.x[op.rs1] ^ int64(op.imm)
case 0b110: // ORI
cpu.x[op.rd] = cpu.x[op.rs1] | int64(op.imm)
case 0b111: // ANDI
cpu.x[op.rd] = cpu.x[op.rs1] & int64(op.imm)
case 0b001: // SLLI
if op.imm>>6 != 0 {
panic(fmt.Sprintf("nyi - invalid instruction %x", instr))
}
cpu.x[op.rd] = cpu.x[op.rs1] << op.imm
case 0b101:
switch op.imm >> 6 {
case 0: // SRLI
cpu.x[op.rd] = int64(uint64(cpu.x[op.rs1]) >> (op.imm & 0b111111))
case 0b010000: // SRAI
cpu.x[op.rd] = cpu.x[op.rs1] >> (op.imm & 0b111111)
default:
panic(fmt.Sprintf("nyi - invalid instruction %x", instr))
}
default:
panic(fmt.Sprintf("nyi - invalid instruction %x", instr))
}
case 0b0110011:
op := parseR(instr)
switch op.funct3 {
case 0b000:
switch op.funct7 {
case 0b0000000: // ADD
cpu.x[op.rd] = cpu.x[op.rs1] + cpu.x[op.rs2]
case 0b0100000: // SUB
cpu.x[op.rd] = cpu.x[op.rs1] - cpu.x[op.rs2]
case 0b0000001: // MUL
cpu.x[op.rd] = cpu.x[op.rs1] * cpu.x[op.rs2]
default:
panic(fmt.Sprintf("nyi - invalid instruction %x", instr))
}
case 0b001:
switch op.funct7 {
case 0: // SLL
cpu.x[op.rd] = cpu.x[op.rs1] << (cpu.x[op.rs2] & 0b111111)
case 1: // MULH
panic("nyi - MULH")
default:
panic(fmt.Sprintf("nyi - invalid instruction %x", instr))
}
case 0b010:
switch op.funct7 {
case 0: // SLT
if cpu.x[op.rs1] < cpu.x[op.rs2] {
cpu.x[op.rd] = 1
} else {
cpu.x[op.rd] = 0
}
case 1: // MULHSU
panic("nyi - MULHSU")
default:
panic(fmt.Sprintf("nyi - invalid instruction %x", instr))
}
case 0b011:
switch op.funct7 {
case 0: // SLTU
if uint64(cpu.x[op.rs1]) < uint64(cpu.x[op.rs2]) {
cpu.x[op.rd] = 1
} else {
cpu.x[op.rd] = 0
}
case 1: // MULHU
a := uint64(cpu.x[op.rs1])
b := uint64(cpu.x[op.rs2])
alo := a & 0xffffffff
ahi := (a >> 32) & 0xffffffff
blo := b & 0xffffffff
bhi := (b >> 32) & 0xffffffff
axbhi := ahi * bhi
axbmid := ahi * blo
bxamid := alo * bhi
axblo := alo * blo
carry := (uint64(uint32(axbmid)) + uint64(uint32(bxamid)) + axblo>>32) >> 32
cpu.x[op.rd] = int64(axbhi + axbmid>>32 + bxamid>>32 + carry)
default:
panic(fmt.Sprintf("nyi - invalid instruction %x", instr))
}
case 0b100:
switch op.funct7 {
case 0: // XOR
cpu.x[op.rd] = cpu.x[op.rs1] ^ cpu.x[op.rs2]
case 1: // DIV
op := parseR(instr)
a1 := cpu.x[op.rs1]
a2 := cpu.x[op.rs2]
if a2 == 0 {
cpu.x[op.rd] = -1
} else if a1 == math.MinInt64 && a2 == -1 {
cpu.x[op.rd] = a1
} else {
cpu.x[op.rd] = a1 / a2
}
default:
panic(fmt.Sprintf("nyi - invalid instruction %x", instr))
}
case 0b101:
switch op.funct7 {
case 0: // SRL
cpu.x[op.rd] = int64(uint64(cpu.x[op.rs1]) >> (cpu.x[op.rs2] & 0b111111))
case 0b0100000: // SRA
cpu.x[op.rd] = cpu.x[op.rs1] >> (cpu.x[op.rs2] & 0b111111)
case 1: // DIVU
op := parseR(instr)
a1 := uint64(cpu.x[op.rs1])
a2 := uint64(cpu.x[op.rs2])
if a2 == 0 {
cpu.x[op.rd] = -1
} else {
cpu.x[op.rd] = int64(a1 / a2)
}
default:
panic(fmt.Sprintf("nyi - invalid instruction %x", instr))
}
case 0b110:
switch op.funct7 {
case 0: // OR
cpu.x[op.rd] = cpu.x[op.rs1] | cpu.x[op.rs2]
case 1: // REM
op := parseR(instr)
a1 := cpu.x[op.rs1]
a2 := cpu.x[op.rs2]
if a2 == 0 {
cpu.x[op.rd] = a1
} else if a1 == math.MinInt64 && a2 == -1 {
cpu.x[op.rd] = 0
} else {
cpu.x[op.rd] = a1 % a2
}
default:
panic(fmt.Sprintf("nyi - invalid instruction %x", instr))
}
case 0b111:
switch op.funct7 {
case 0: // AND
cpu.x[op.rd] = cpu.x[op.rs1] & cpu.x[op.rs2]
case 1: // REMU
op := parseR(instr)
a1 := uint64(cpu.x[op.rs1])
a2 := uint64(cpu.x[op.rs2])
if a2 == 0 {
cpu.x[op.rd] = int64(a1)
} else {
cpu.x[op.rd] = int64(a1 % a2)
}
default:
panic(fmt.Sprintf("nyi - invalid instruction %x", instr))
}
default:
panic(fmt.Sprintf("nyi - invalid instruction %x", instr))
}
case 0b0001111: // FENCE/FENCE.I
// TODO: Is it okay to do nothing?
case 0b1110011:
op := parseCSR(instr)
switch op.funct3 {
case 0b000:
if op.rd != 0 {
panic(fmt.Sprintf("nyi - invalid instruction %x", instr))
}
switch op.csr {
case 0: // ECALL
switch cpu.priv {
case User:
return false, Trap{reason: EnvironmentCallFromUMode, value: addr}
case Supervisor:
return false, Trap{reason: EnvironmentCallFromSMode, value: addr}
case Hypervisor:
return false, Trap{reason: EnvironmentCallFromHMode, value: addr}
case Machine:
return false, Trap{reason: EnvironmentCallFromMMode, value: addr}
default:
panic("invalid CPU privilege")
}
case 1: // EBREAK
return false, Trap{reason: Breakpoint, value: addr}
case 0b000000000010: // URET
panic("nyi - URET")
case 0b000100000010: // SRET
cpu.pc = cpu.readcsr(SEPC)
status := cpu.readcsr(SSTATUS)
cpu.priv = Privilege((status >> 8) & 0b1)
spie := (status >> 5) & 0b1
var mpriv uint64
if cpu.priv == Machine {
mpriv = (status >> 17) & 0b1
}
status = status&^0x20122 | mpriv<<17 | spie<<1 | 1<<5
cpu.writecsr(SSTATUS, status)
case 0b001100000010: // MRET
cpu.pc = cpu.readcsr(MEPC)
status := cpu.readcsr(MSTATUS)
cpu.priv = Privilege((status >> 11) & 0b11)
mpie := (status >> 7) & 0b1
var mpriv uint64
if cpu.priv == Machine {
mpriv = (status >> 17) & 0b1
}
status = status&^0x21888 | mpriv<<17 | mpie<<3 | 1<<7
cpu.writecsr(MSTATUS, status)
case 0b000100000101: // WFI
cpu.wfi = true
default:
switch op.csr >> 5 {
case 0b0001001: // SFENCE.VMA
// TODO: Is it okat to do nothing?
case 0b0010001:
panic("nyi - HFENCE.BVMA")
case 0b1010001:
panic("nyi - HFENCE.GVMA")
default:
panic(fmt.Sprintf("nyi - invalid instruction %x", instr))
}
}
case 0b001: // CSRRW
t := cpu.readcsr(uint16(op.csr))
cpu.writecsr(uint16(op.csr), uint64(cpu.x[op.rs]))
cpu.x[op.rd] = int64(t)
case 0b010: // CSRRS
t := cpu.readcsr(uint16(op.csr))
cpu.writecsr(uint16(op.csr), t|uint64(cpu.x[op.rs]))
cpu.x[op.rd] = int64(t)
case 0b011: // CSRRC
t := cpu.readcsr(uint16(op.csr))
trs := cpu.x[op.rs]
cpu.x[op.rd] = int64(t)
cpu.writecsr(uint16(op.csr), uint64(cpu.x[op.rd] & ^trs))
case 0b101: // CSRRWI
t := cpu.readcsr(uint16(op.csr))
cpu.x[op.rd] = int64(t)
cpu.writecsr(uint16(op.csr), uint64(op.rs))
case 0b110: // CSRRSI
t := cpu.readcsr(uint16(op.csr))
cpu.x[op.rd] = int64(t)
cpu.writecsr(uint16(op.csr), uint64(cpu.x[op.rd]|int64(op.rs)))
case 0b111: // CSRRCI
t := cpu.readcsr(uint16(op.csr))
cpu.x[op.rd] = int64(t)
cpu.writecsr(uint16(op.csr), uint64(cpu.x[op.rd] & ^int64(op.rs)))
default:
panic(fmt.Sprintf("nyi - invalid instruction %x", instr))
}
case 0b0111011:
op := parseR(instr)
switch op.funct3 {
case 0b000:
switch op.funct7 {
case 0b0000000: // ADD
cpu.x[op.rd] = int64(int32(cpu.x[op.rs1]) + int32(cpu.x[op.rs2]))
case 0b0100000: // SUB
cpu.x[op.rd] = int64(int32(cpu.x[op.rs1]) - int32(cpu.x[op.rs2]))
case 1: // MULW
cpu.x[op.rd] = int64(int32(cpu.x[op.rs1]) * int32(cpu.x[op.rs2]))
default:
panic(fmt.Sprintf("nyi - invalid instruction %x", instr))
}
case 0b001:
switch op.funct7 {
case 0:
cpu.x[op.rd] = int64(int32(cpu.x[op.rs1]) << (cpu.x[op.rs2] & 0b11111))
default:
panic(fmt.Sprintf("nyi - invalid instruction %x", instr))
}
case 0b100:
switch op.funct7 {
case 1: // DIVW
op := parseR(instr)
a1 := int32(cpu.x[op.rs1])
a2 := int32(cpu.x[op.rs2])
if a2 == 0 {
cpu.x[op.rd] = -1
} else if a1 == math.MinInt32 && a2 == -1 {
cpu.x[op.rd] = int64(a1)
} else {
cpu.x[op.rd] = int64(a1 / a2)
}
default:
panic(fmt.Sprintf("nyi - invalid instruction %x", instr))
}
case 0b101:
switch op.funct7 {
case 0: // SRL
cpu.x[op.rd] = int64(int32(uint32(uint64(cpu.x[op.rs1])) >> (cpu.x[op.rs2] & 0b11111)))
case 0b0100000: // SRA
cpu.x[op.rd] = int64(int32(cpu.x[op.rs1]) >> (cpu.x[op.rs2] & 0b11111))
case 1: // DIVUW
op := parseR(instr)
a1 := uint32(cpu.x[op.rs1])
a2 := uint32(cpu.x[op.rs2])
if a2 == 0 {
cpu.x[op.rd] = -1
} else {
cpu.x[op.rd] = int64(int32(a1 / a2))
}
default:
panic(fmt.Sprintf("nyi - invalid instruction %x", instr))
}
case 0b110:
switch op.funct7 {
case 1: // REMW
op := parseR(instr)
a1 := int32(cpu.x[op.rs1])
a2 := int32(cpu.x[op.rs2])
if a2 == 0 {
cpu.x[op.rd] = int64(a1)
} else if a1 == math.MinInt32 && a2 == -1 {
cpu.x[op.rd] = 0
} else {
cpu.x[op.rd] = int64(a1 % a2)
}
default:
panic(fmt.Sprintf("nyi - invalid instruction %x", instr))
}
case 0b111:
switch op.funct7 {
case 1: // REMUW
op := parseR(instr)
a1 := uint32(cpu.x[op.rs1])
a2 := uint32(cpu.x[op.rs2])
if a2 == 0 {
cpu.x[op.rd] = int64(int32(a1))
} else {
cpu.x[op.rd] = int64(int32(a1 % a2))
}
default:
panic(fmt.Sprintf("nyi - invalid instruction %x", instr))
}
default:
panic("nyi - 0111011")
}
case 0b0101111:
op := parseR(instr)
switch op.funct7 >> 2 {
case 0b00010:
switch op.funct3 {
case 0b010: // LR.W
v, ok, trap := cpu.readuint32(uint64(cpu.x[op.rs1]))
if !ok {
return false, trap
}
cpu.reservationSet = true
cpu.reservation = uint64(cpu.x[op.rs1])
cpu.x[op.rd] = int64(int32(v))
case 0b011: // LR.D
v, ok, trap := cpu.readuint64(uint64(cpu.x[op.rs1]))
if !ok {
return false, trap
}
cpu.reservationSet = true
cpu.reservation = uint64(cpu.x[op.rs1])
cpu.x[op.rd] = int64(v)
default:
panic(fmt.Sprintf("nyi - invalid instruction %x", instr))
}
case 0b00011:
switch op.funct3 {
case 0b010: // SC.W
if cpu.reservationSet && cpu.reservation == uint64(cpu.x[op.rs1]) {
ok, trap := cpu.writeuint32(uint64(cpu.x[op.rs1]), uint32(cpu.x[op.rs2]))
if !ok {
return false, trap
}
cpu.reservationSet = false
cpu.x[op.rd] = 0
} else {
cpu.x[op.rd] = 1
}
case 0b011: // SC.D
if cpu.reservationSet && cpu.reservation == uint64(cpu.x[op.rs1]) {
ok, trap := cpu.writeuint64(uint64(cpu.x[op.rs1]), uint64(cpu.x[op.rs2]))
if !ok {
return false, trap
}
cpu.reservationSet = false
cpu.x[op.rd] = 0
} else {
cpu.x[op.rd] = 1
}
default:
panic(fmt.Sprintf("nyi - invalid instruction %x", instr))
}
case 0b00001:
switch op.funct3 {
case 0b010: // AMOSWAP.W
v, ok, trap := cpu.readuint32(uint64(cpu.x[op.rs1]))
if !ok {
return false, trap
}
ok, trap = cpu.writeuint32(uint64(cpu.x[op.rs1]), uint32(cpu.x[op.rs2]))
if !ok {
return false, trap
}
cpu.x[op.rd] = int64(int32(v))
case 0b011: // AMOSWAP.D
v, ok, trap := cpu.readuint64(uint64(cpu.x[op.rs1]))
if !ok {
return false, trap
}
ok, trap = cpu.writeuint64(uint64(cpu.x[op.rs1]), uint64(cpu.x[op.rs2]))
if !ok {
return false, trap
}
cpu.x[op.rd] = int64(v)
default:
panic(fmt.Sprintf("nyi - invalid instruction %x", instr))
}
case 0b00000:
switch op.funct3 {
case 0b010: // AMOADD.W
v, ok, trap := cpu.readuint32(uint64(cpu.x[op.rs1]))
if !ok {
return false, trap
}
ok, trap = cpu.writeuint32(uint64(cpu.x[op.rs1]), uint32(cpu.x[op.rs2]+int64(int32(v))))
if !ok {
return false, trap
}
cpu.x[op.rd] = int64(int32(v))
case 0b011: // AMOADD.D
v, ok, trap := cpu.readuint64(uint64(cpu.x[op.rs1]))
if !ok {
return false, trap
}
ok, trap = cpu.writeuint64(uint64(cpu.x[op.rs1]), uint64(cpu.x[op.rs2]+int64(v)))
if !ok {
return false, trap
}
cpu.x[op.rd] = int64(v)
default:
panic(fmt.Sprintf("nyi - invalid instruction %x", instr))
}
case 0b00100:
panic("nyi - AMOXOR.W")
case 0b01100:
switch op.funct3 {
case 0b010: // AMOAND.W
v, ok, trap := cpu.readuint32(uint64(cpu.x[op.rs1]))
if !ok {
return false, trap
}
ok, trap = cpu.writeuint32(uint64(cpu.x[op.rs1]), uint32(cpu.x[op.rs2]&int64(int32(v))))
if !ok {
return false, trap
}
cpu.x[op.rd] = int64(int32(v))
case 0b011: // AMOAND.D
v, ok, trap := cpu.readuint64(uint64(cpu.x[op.rs1]))
if !ok {
return false, trap
}
ok, trap = cpu.writeuint64(uint64(cpu.x[op.rs1]), uint64(cpu.x[op.rs2]&int64(v)))
if !ok {
return false, trap
}
cpu.x[op.rd] = int64(v)
default:
panic(fmt.Sprintf("nyi - invalid instruction %x", instr))
}
case 0b01000:
switch op.funct3 {
case 0b010: // AMOOR.W
v, ok, trap := cpu.readuint32(uint64(cpu.x[op.rs1]))
if !ok {
return false, trap
}
ok, trap = cpu.writeuint32(uint64(cpu.x[op.rs1]), uint32(cpu.x[op.rs2]|int64(int32(v))))
if !ok {
return false, trap
}
cpu.x[op.rd] = int64(int32(v))
case 0b011: // AMOOR.D
v, ok, trap := cpu.readuint64(uint64(cpu.x[op.rs1]))
if !ok {
return false, trap
}
ok, trap = cpu.writeuint64(uint64(cpu.x[op.rs1]), uint64(cpu.x[op.rs2]|int64(v)))
if !ok {
return false, trap
}
cpu.x[op.rd] = int64(v)
default:
panic(fmt.Sprintf("nyi - invalid instruction %x", instr))
}
case 0b10000:
switch op.funct3 {
case 0b010: // AMOMIN.W
v, ok, trap := cpu.readuint32(uint64(cpu.x[op.rs1]))
if !ok {
return false, trap
}
min := v
if int32(cpu.x[op.rs2]) < int32(min) {
min = uint32(cpu.x[op.rs2])
}
ok, trap = cpu.writeuint32(uint64(cpu.x[op.rs1]), min)
if !ok {
return false, trap
}
cpu.x[op.rd] = int64(int32(v))
case 0b011: // AMOMIN.D
v, ok, trap := cpu.readuint64(uint64(cpu.x[op.rs1]))
if !ok {
return false, trap
}
min := v
if cpu.x[op.rs2] < int64(min) {
min = uint64(cpu.x[op.rs2])
}
ok, trap = cpu.writeuint64(uint64(cpu.x[op.rs1]), min)
if !ok {
return false, trap
}
cpu.x[op.rd] = int64(v)
}
case 0b10100:
switch op.funct3 {
case 0b010: // AMOMAX.W
v, ok, trap := cpu.readuint32(uint64(cpu.x[op.rs1]))
if !ok {
return false, trap
}
max := v
if int32(cpu.x[op.rs2]) > int32(max) {
max = uint32(cpu.x[op.rs2])
}
ok, trap = cpu.writeuint32(uint64(cpu.x[op.rs1]), max)
if !ok {
return false, trap
}
cpu.x[op.rd] = int64(int32(v))
case 0b011: // AMOMAX.D
v, ok, trap := cpu.readuint64(uint64(cpu.x[op.rs1]))
if !ok {
return false, trap
}
max := v
if cpu.x[op.rs2] > int64(max) {
max = uint64(cpu.x[op.rs2])
}
ok, trap = cpu.writeuint64(uint64(cpu.x[op.rs1]), max)
if !ok {
return false, trap
}
cpu.x[op.rd] = int64(v)
}
case 0b11000:
switch op.funct3 {
case 0b010: // AMOMINU.W
v, ok, trap := cpu.readuint32(uint64(cpu.x[op.rs1]))
if !ok {
return false, trap
}
min := v
if uint32(cpu.x[op.rs2]) < min {
min = uint32(cpu.x[op.rs2])
}
ok, trap = cpu.writeuint32(uint64(cpu.x[op.rs1]), min)
if !ok {
return false, trap
}
cpu.x[op.rd] = int64(int32(v))
case 0b011: // AMOMINU.D
v, ok, trap := cpu.readuint64(uint64(cpu.x[op.rs1]))
if !ok {
return false, trap
}
min := v
if uint64(cpu.x[op.rs2]) < min {
min = uint64(cpu.x[op.rs2])
}
ok, trap = cpu.writeuint64(uint64(cpu.x[op.rs1]), min)
if !ok {
return false, trap
}
cpu.x[op.rd] = int64(v)
}
case 0b11100:
switch op.funct3 {
case 0b010: // AMOMAXU.W
v, ok, trap := cpu.readuint32(uint64(cpu.x[op.rs1]))
if !ok {
return false, trap
}
max := v
if uint32(cpu.x[op.rs2]) > max {
max = uint32(cpu.x[op.rs2])
}
ok, trap = cpu.writeuint32(uint64(cpu.x[op.rs1]), max)
if !ok {
return false, trap
}
cpu.x[op.rd] = int64(int32(v))
case 0b011: // AMOMAXU.D
v, ok, trap := cpu.readuint64(uint64(cpu.x[op.rs1]))
if !ok {
return false, trap
}
max := v
if uint64(cpu.x[op.rs2]) > max {
max = uint64(cpu.x[op.rs2])
}
ok, trap = cpu.writeuint64(uint64(cpu.x[op.rs1]), max)
if !ok {
return false, trap
}
cpu.x[op.rd] = int64(v)
}
default:
panic("nyi - atomic")
}
case 0b0000111: // FLW
op := parseI(instr)
v, ok, trap := cpu.readuint32(uint64(cpu.x[op.rs1] + int64(op.imm)))
if !ok {
return false, trap
}
cpu.f[op.rd] = math.Float64frombits(uint64(int64(int32(v))))
case 0b0100111: // FSW
op := parseS(instr)
v := uint32(math.Float64bits(cpu.f[op.rs2]))
ok, trap := cpu.writeuint32(uint64(cpu.x[op.rs1]+int64(op.imm)), v)
if !ok {
return false, trap
}
case 0b1000011:
panic("nyi - FMADD.S")
case 0b1000111:
panic("nyi - FMSUB.S")
case 0b1001011:
panic("nyi - FNMSUB.S")
case 0b1001111:
panic("nyi - FNMADD.S")
case 0b1010011:
op := parseR(instr)
switch op.funct7 {
case 0b0000000:
panic("nyi - FADD.S")
case 0b0000100:
panic("nyi - FSUB.S")
case 0b0001000:
panic("nyi - FMUL.S")
case 0b0001100:
panic("nyi - FDIV.S")
case 0b0101100:
panic("nyi - FSQRT.S")
case 0b0010000:
panic("nyi - FSGN*.S")
case 0b0010100:
panic("nyi - FM**.S")
case 0b1100000:
op := parseR(instr)
switch op.rs2 {
case 0b00000: // FCVT.W.S
f64 := cpu.f[op.rs1]
f32 := float32(f64)
i32 := int32(f32)
cpu.x[op.rd] = int64(i32)
case 0b00001: // FCVT.WU.S
cpu.x[op.rd] = int64(uint64(uint32(float32(cpu.f[op.rs1]))))
default:
panic(fmt.Sprintf("invalid - %x", instr))
}
case 0b1110000: // FMV.X.W
op := parseR(instr)
bits := math.Float32bits(float32(cpu.f[op.rs1]))
ibits := int32(bits)
cpu.x[op.rd] = int64(ibits)
case 0b1010000:
panic("nyi - FEQ.S/*")
case 0b1101000:
op := parseR(instr)
switch op.rs2 {
case 0b00000: // FCVT.S.W
cpu.f[op.rd] = float64(float32(int32(cpu.x[op.rs1])))
case 0b00001: // FCVT.S.WU
cpu.f[op.rd] = float64(float32(uint32(cpu.x[op.rs1])))
case 0b00010: // FCVT.S.L
cpu.f[op.rd] = float64(cpu.x[op.rs1])
case 0b00011: // FCVT.S.LU
cpu.f[op.rd] = float64(uint64(cpu.x[op.rs1]))
default:
panic(fmt.Sprintf("invalid - %x", instr))
}
case 0b1111000: // FMV.W.X
op := parseR(instr)
cpu.f[op.rd] = math.Float64frombits(uint64(uint32(cpu.x[op.rs1])))
case 0b0000001:
panic("nyi - FADD.D")
case 0b0000101:
panic("nyi - FSUB.D")
case 0b0001001:
panic("nyi - FMUL.D")
case 0b0001101:
panic("nyi - FDIV.D")
case 0b0101101:
panic("nyi - FSQRT.D")
case 0b0010001:
panic("nyi - FSGN*.D")
case 0b0010101:
panic("nyi - FM**.D")
case 0b1100001:
op := parseR(instr)
switch op.rs2 {
case 0b00000: // FCVT.W.D
cpu.x[op.rd] = int64(int32(uint32(cpu.f[op.rs1])))
case 0b00001: // FCVT.WU.D
cpu.x[op.rd] = int64(uint64(uint32(cpu.f[op.rs1])))
default:
panic(fmt.Sprintf("invalid - %x", instr))
}
case 0b1110001:
panic("nyi - FMV.X.D")
case 0b1010001:
panic("nyi - FEQ.D/*")
case 0b1101001:
op := parseR(instr)
switch op.rs2 {
case 0b00000: // FCVT.D.W
cpu.f[op.rd] = float64(int32(cpu.x[op.rs1]))
case 0b00001: // FCVT.D.WU
cpu.f[op.rd] = float64(uint32(cpu.x[op.rs1]))
default:
panic(fmt.Sprintf("invalid - %x", instr))
}
default:
panic(fmt.Sprintf("invalid - %x", instr))
}
case 0b0011011:
op := parseI(instr)
switch op.funct3 {
case 0b000: // ADDIW
cpu.x[op.rd] = int64(int32(cpu.x[op.rs1] + int64(op.imm)))
case 0b001: // SLLIW
if op.imm>>5 != 0 {
panic(fmt.Sprintf("nyi - invalid instruction %x", instr))
}
cpu.x[op.rd] = int64(int32(cpu.x[op.rs1] << op.imm))
case 0b101:
switch op.imm >> 6 {
case 0: // SRLIW
cpu.x[op.rd] = int64(int32(uint32(int32(cpu.x[op.rs1])) >> (op.imm & 0b111111)))
case 0b010000: // SRAIW
cpu.x[op.rd] = int64(int32(cpu.x[op.rs1]) >> (op.imm & 0b111111))
default:
panic(fmt.Sprintf("nyi - invalid instruction %x", instr))
}
default:
panic(fmt.Sprintf("nyi - invalid instruction %x", instr))
}
default:
panic(fmt.Sprintf("nyi - opcode %x", instr&0x7f))
}
return true, Trap{}
}
func (cpu *CPU) readcsr(csr uint16) uint64 {
switch csr {
case FFLAGS:
return cpu.readcsr(FCSR) & 0x1f
case FRM:
return (cpu.readcsr(FCSR) >> 5) & 0x7
case SSTATUS:
return cpu.readcsr(MSTATUS) & 0x80000003000de162
case SIE:
return cpu.csr[MIE] & 0x222
case SIP:
return cpu.csr[MIP] & 0x222
case TIME:
return cpu.clint.mtime
case MSTATUS:
// Force UXL and SXL to RV64
return cpu.csr[MSTATUS]&^0xF00000000 | 0xA00000000
case MISA:
// A, B, C, D, F, I, M, N, S, U + RV64 +
return 0x800000008014312f
}
return cpu.csr[csr]
}
func (cpu *CPU) writecsr(csr uint16, v uint64) {
switch csr {
case FFLAGS:
cpu.csr[FCSR] = cpu.csr[FCSR]&^0x1f | v&0x1f
case FRM:
cpu.csr[FCSR] = cpu.csr[FCSR]&^0xe0 | (v<<5)&0xe0
case SSTATUS:
// if (cpu.csr[MSTATUS]>>1)&1 != (v>>1)&1 {
// fmt.Fprintf(os.Stderr, "sie = %d\n", (v>>1)&1)
// }
cpu.csr[MSTATUS] = cpu.csr[MSTATUS]&^0x80000003000de162 | v&0x80000003000de162
case SIE:
cpu.csr[MIE] = cpu.csr[MIE]&^0x222 | v&0x222
case SIP:
cpu.csr[MIP] = cpu.csr[MIP]&^0x222 | v&0x222
case TIME:
cpu.clint.mtime = v
case MIDELEG:
cpu.csr[csr] = v & 0x666
case SATP:
switch v >> 60 {
case 0, 8, 9:
cpu.mode = AddressMode(v >> 60)
default:
panic("invalid addressing mode")
}
cpu.csr[csr] = v
default:
cpu.csr[csr] = v
}
}
func (cpu *CPU) virtualToPhysical(vaddr uint64, access Access) (uint64, bool) {
switch cpu.mode {
case None:
return vaddr, true
case SV39:
switch cpu.priv {
case Machine:
mstatus := cpu.readcsr(MSTATUS)
if access == Execute {
return vaddr, true
}
if (mstatus>>17)&1 == 0 {
return vaddr, true
}
priv := Privilege((mstatus >> 9) & 3)
if priv == Machine {
return vaddr, true
}
currentPriv := cpu.priv
cpu.priv = priv
v, ok := cpu.virtualToPhysical(vaddr, access)
cpu.priv = currentPriv
return v, ok
case User, Supervisor:
rootppn := cpu.readcsr(SATP) & 0xfffffffffff
vpns := []uint64{(vaddr >> 12) & 0x1ff, (vaddr >> 21) & 0x1ff, (vaddr >> 30) & 0x1ff}
paddr, ok := cpu.walkPageTables(vaddr, 3-1, rootppn, vpns, access)
if !ok {
return 0, false
}
return paddr, true
default:
panic("invalid CPU priv")
}
default:
panic("invalid addressing mode")
}
}
func (cpu *CPU) walkPageTables(vaddr uint64, level uint8, parentppn uint64, vpns []uint64, access Access) (uint64, bool) {
// fmt.Printf("walkPageTables: %x, %d, %x, %x\n", vaddr, level, parentppn, vpns)
pagesize := uint64(4096)
ptesize := uint64(8)
pteaddr := parentppn*pagesize + vpns[level]*ptesize
pte := cpu.readphysicaluint64(pteaddr)
ppn := (pte >> 10) & 0xfffffffffff
ppns := []uint64{(pte >> 10) & 0x1ff, (pte >> 19) & 0x1ff, (pte >> 28) & 0x3ffffff}
// rsw := (pte >> 8) & 0b11
d := (pte >> 7) & 0b1
a := (pte >> 6) & 0b1
// g := (pte >> 5) & 0b1
// u := (pte >> 4) & 0b1
x := (pte >> 3) & 0b1
w := (pte >> 2) & 0b1
r := (pte >> 1) & 0b1
v := (pte >> 0) & 0b1
if v == 0 || (r == 0 && w == 1) { // Not valid, or invalid write-only
return 0, false
}
if r == 0 && x == 0 {
if level == 0 { // pointer to page table entry at leaf layer
return 0, false
}
return cpu.walkPageTables(vaddr, level-1, ppn, vpns, access)
}
if a == 0 || (access == Write && d == 0) {
newpte := pte | 1<<6
if access == Write {
newpte |= 1 << 7
}
cpu.writephysical(pteaddr, uint8(newpte))
cpu.writephysical(pteaddr+1, uint8(newpte>>8))
cpu.writephysical(pteaddr+2, uint8(newpte>>16))
cpu.writephysical(pteaddr+3, uint8(newpte>>24))
cpu.writephysical(pteaddr+4, uint8(newpte>>32))
cpu.writephysical(pteaddr+5, uint8(newpte>>40))
cpu.writephysical(pteaddr+6, uint8(newpte>>48))
cpu.writephysical(pteaddr+7, uint8(newpte>>56))
}
if (access == Execute && x == 0) || (access == Read && r == 0) || (access == Write && w == 0) {
return 0, false
}
offset := vaddr & 0xfff
if level == 2 {
if ppns[1] != 0 || ppns[0] != 0 {
return 0, false
}
return ppns[2]<<30 | vpns[1]<<21 | vpns[0]<<12 | offset, true
} else if level == 1 {
if ppns[0] != 0 {
return 0, false
}
return ppns[2]<<30 | ppns[1]<<21 | vpns[0]<<12 | offset, true
} else if level == 0 {
return ppn<<12 | offset, true
}
panic("invalid level")
}
func (cpu *CPU) readraw(vaddr uint64) (uint8, bool) {
paddr, ok := cpu.virtualToPhysical(vaddr, Read)
if !ok {
return 0, false
}
return cpu.readphysical(paddr), true
}
func (cpu *CPU) readphysical(addr uint64) (ret uint8) {
if addr >= MEMORYBASE {
return cpu.mem[addr-MEMORYBASE]
}
if addr >= 0x00001020 && addr <= 0x00001fff {
daddr := addr - 0x00001020
if daddr >= uint64(len(dtb)) {
return 0
}
return dtb[daddr]
}
if addr >= 0x02000000 && addr <= 0x0200ffff {
return cpu.clint.readuint8(addr)
}
if addr >= 0x0C000000 && addr <= 0x0fffffff {
return cpu.plic.readuint8(addr)
}
if addr >= 0x10000000 && addr <= 0x100000ff {
return cpu.uart.readuint8(addr)
}
if addr >= 0x10001000 && addr <= 0x10001fff {
return cpu.disk.readuint8(addr)
}
panic(fmt.Sprintf("nyi - unsupported address %x", addr))
}
func (cpu *CPU) readphysicaluint64(addr uint64) uint64 {
val := uint64(0)
if addr >= MEMORYBASE && addr%8 == 0 { // Read directly from RAM
memaddr := addr - MEMORYBASE
val = cpu.mem64[memaddr/8]
} else {
for i := uint64(0); i < 8; i++ {
val |= uint64(cpu.readphysical(addr)) << (i * 8)
}
}
return val
}
func (cpu *CPU) writeraw(vaddr uint64, v byte) bool {
paddr, ok := cpu.virtualToPhysical(vaddr, Write)
if !ok {
return false
}
cpu.writephysical(paddr, v)
return true
}
func (cpu *CPU) writephysical(addr uint64, v byte) {
if addr >= MEMORYBASE {
cpu.mem[addr-MEMORYBASE] = v
return
}
if addr >= 0x00001020 && addr <= 0x00001fff {
panic("nyi - cannot write to dtb")
}
if addr >= 0x02000000 && addr <= 0x0200ffff {
cpu.clint.writeuint8(addr, v)
return
}
if addr >= 0x0C000000 && addr <= 0x0fffffff {
cpu.plic.writeuint8(addr, v)
return
}
if addr >= 0x10000000 && addr <= 0x100000ff {
cpu.uart.writeuint8(addr, v)
return
}
if addr >= 0x10001000 && addr <= 0x10001FFF {
cpu.disk.writeuint8(addr, v)
return
}
panic(fmt.Sprintf("nyi - unsupported address %x: %x", addr, v))
}
func (cpu *CPU) readuint64(addr uint64) (uint64, bool, Trap) {
val := uint64(0)
if addr&0xfff <= 0x1000-4 { // instruction is within a single page
paddr, ok := cpu.virtualToPhysical(addr, Read)
if !ok {
return 0, false, Trap{reason: LoadPageFault, value: addr}
}
for i := uint64(0); i < 8; i++ {
x := cpu.readphysical(paddr + i)
val |= uint64(x) << (i * 8)
}
} else { // instruction is across two pages
for i := uint64(0); i < 8; i++ {
paddr, ok := cpu.virtualToPhysical(addr+i, Execute)
if !ok {
return 0, false, Trap{reason: LoadPageFault, value: addr + i}
}
x := cpu.readphysical(paddr)
val |= uint64(x) << (i * 8)
}
}
return val, true, Trap{}
}
func (cpu *CPU) readuint32(addr uint64) (uint32, bool, Trap) {
val := uint32(0)
for i := uint64(0); i < 4; i++ {
x, ok := cpu.readraw(addr + i)
if !ok {
return 0, false, Trap{reason: LoadPageFault, value: addr + i}
}
val |= uint32(x) << (i * 8)
}
return val, true, Trap{}
}
func (cpu *CPU) readuint16(addr uint64) (uint16, bool, Trap) {
val := uint16(0)
for i := uint64(0); i < 2; i++ {
x, ok := cpu.readraw(addr + i)
if !ok {
return 0, false, Trap{reason: LoadPageFault, value: addr + i}
}
val |= uint16(x) << (i * 8)
}
return val, true, Trap{}
}
func (cpu *CPU) readuint8(addr uint64) (uint8, bool, Trap) {
x, ok := cpu.readraw(addr)
if !ok {
return 0, false, Trap{reason: LoadPageFault, value: addr}
}
return x, true, Trap{}
}
func (cpu *CPU) writeuint64(addr uint64, val uint64) (bool, Trap) {
if addr&0xfff <= 0x1000-8 { // uint64 is within a single page
paddr, ok := cpu.virtualToPhysical(addr, Write)
if !ok {
return false, Trap{reason: StorePageFault, value: addr}
}
if paddr >= MEMORYBASE && (paddr-MEMORYBASE)%8 == 0 {
cpu.mem64[(paddr-MEMORYBASE)/8] = val
} else {
for i := uint64(0); i < 8; i++ {
cpu.writephysical(paddr+i, byte(val>>(i*8)))
}
}
return true, Trap{}
} else { // uint64 is across two pages
for i := uint64(0); i < 8; i++ {
paddr, ok := cpu.virtualToPhysical(addr+i, Write)
if !ok {
return false, Trap{reason: StorePageFault, value: addr + i}
}
cpu.writephysical(paddr, byte(val>>(i*8)))
}
return true, Trap{}
}
}
func (cpu *CPU) writeuint32(addr uint64, val uint32) (bool, Trap) {
for i := uint64(0); i < 4; i++ {
ok := cpu.writeraw(addr+i, byte(val>>(i*8)))
if !ok {
return false, Trap{reason: StorePageFault, value: addr + i}
}
}
return true, Trap{}
}
func (cpu *CPU) writeuint16(addr uint64, val uint16) (bool, Trap) {
for i := uint64(0); i < 2; i++ {
ok := cpu.writeraw(addr+i, byte(val>>(i*8)))
if !ok {
return false, Trap{reason: StorePageFault, value: addr + i}
}
}
return true, Trap{}
}
func (cpu *CPU) writeuint8(addr uint64, val uint8) (bool, Trap) {
ok := cpu.writeraw(addr, byte(val))
if !ok {
return false, Trap{reason: StorePageFault, value: addr}
}
return true, Trap{}
}
func (cpu *CPU) decompress(instr uint32) uint32 {
op := instr & 0b11
funct3 := (instr >> 13) & 0b111
switch op {
case 0b00:
switch funct3 {
case 0b000:
rd := (instr >> 2) & 0x7
nzuimm := (instr>>7)&0x30 | (instr>>1)&0x3c0 | (instr>>4)&0x4 | (instr>>2)&0x8
if nzuimm != 0 { // C.ADDI4SPN = addi rd+8, x2, nzuimm
return nzuimm<<20 | 2<<15 | (rd+8)<<7 | 0x13
} else {
panic("reserved")
}
case 0b001: // C.FLD = fld rd+8, offset(rs1+8)
rs1 := (instr >> 7) & 0x7
rd := (instr >> 2) & 0x7
offset := (instr>>7)&0x38 | (instr<<1)&0xc0
return offset<<20 | (rs1+8)<<15 | 3<<12 | (rd+8)<<7 | 0x7
case 0b010: // C.LW = lw rd+8, offset(rs1+8)
rs1 := (instr >> 7) & 0x7
rd := (instr >> 2) & 0x7
offset := (instr>>7)&0x38 | (instr<<1)&0x40 | (instr>>4)&0x4
return offset<<20 | (rs1+8)<<15 | 2<<12 | (rd+8)<<7 | 0x3
case 0b011: // C.LD = ld rd+8, offset(rs1+8)
rs1 := (instr >> 7) & 0x7
rd := (instr >> 2) & 0x7
offset := (instr>>7)&0x38 | (instr<<1)&0xc0
return offset<<20 | (rs1+8)<<15 | 3<<12 | (rd+8)<<7 | 0x3
case 0b100:
panic("nyi - reserved")
case 0b101: // C.FSD = fsd rs2+8, offset(rs1+8)
rs1 := (instr >> 7) & 0x7
rs2 := (instr >> 2) & 0x7
offset := (instr>>7)&0x38 | (instr<<1)&0xc0
imm115 := (offset >> 5) & 0x7f
imm40 := offset & 0x1f
return imm115<<25 | (rs2+8)<<20 | (rs1+8)<<15 | 3<<12 | imm40<<7 | 0x27
case 0b110: // C.SW = sw rs2+8, offset(rs1+8)
rs1 := (instr >> 7) & 0x7
rs2 := (instr >> 2) & 0x7
offset := (instr>>7)&0x38 | (instr<<1)&0x40 | (instr>>4)&0x4
imm115 := (offset >> 5) & 0x3f
imm40 := offset & 0x1f
return imm115<<25 | (rs2+8)<<20 | (rs1+8)<<15 | 2<<12 | imm40<<7 | 0x23
case 0b111: // C.SD = sd rs2+8, offset(rs1+8)
rs1 := (instr >> 7) & 0x7
rs2 := (instr >> 2) & 0x7
offset := (instr>>7)&0x38 | (instr<<1)&0xc0
imm115 := (offset >> 5) & 0x7f
imm40 := offset & 0x1f
return imm115<<25 | (rs2+8)<<20 | (rs1+8)<<15 | 3<<12 | imm40<<7 | 0x23
default:
panic("unreachable")
}
case 0b01:
switch funct3 {
case 0b000:
r := instr & 0b111110000000
imm := (instr>>7)&0x20 | (instr>>2)&0x1f
if instr&0x1000 != 0 {
imm |= 0xffffffc0
}
if r == 0 && imm == 0 { // C.NOP = addi x0, x0, 0
return 0x13
} else { // C.ADDI = addi r, r, imm
return imm<<20 | r<<8 | r | 0x13
}
case 0b001:
r := instr & 0b111110000000
imm := (instr>>7)&0x20 | (instr>>2)&0x1f
if instr&0x1000 != 0 {
imm |= 0xffffffc0
}
if r != 0 { // C.ADDIW = addiw r, r, imm
return imm<<20 | r<<8 | r | 0x1b
} else {
panic("reserved")
}
case 0b010: // C.LI = addi rd, x0, imm
r := instr & 0b111110000000
imm := (instr>>7)&0x20 | (instr>>2)&0x1f
if instr&0x1000 != 0 {
imm |= 0xffffffc0
}
if r != 0 { // C.LI = addi rd, x0, imm
return imm<<20 | r | 0x13
} else { // hint
panic("nyi - hint")
}
case 0b011:
r := instr & 0b111110000000
if r == 0b100000000 {
imm := (instr>>3)&0x200 | (instr>>2)&0x10 | (instr<<1)&0x40 | (instr<<4)&0x180 | (instr<<3)&0x20
if instr&0x1000 != 0 {
imm |= 0xfffffc00
}
if imm != 0 { // C.ADDI16SP
return imm<<20 | r<<8 | r | 0x13
} else {
panic("reserved")
}
} else if r != 0 {
nzimm := (instr<<5)&0x20000 | (instr<<10)&0x1f000
if instr&0x1000 != 0 {
nzimm |= 0xfffc0000
}
if nzimm != 0 { // C.LUI = lui r, nzimm
return nzimm | r | 0x37
} else {
panic("nyi - reserved")
}
} else {
panic("nyi")
}
case 0b100:
funct2 := (instr >> 10) & 0x3
switch funct2 {
case 0b00: // C.SRLI = srli rs1+8, rs1+8, shamt
rs1 := (instr >> 7) & 0x7
shamt := (instr>>7)&0x20 | (instr>>2)&0x1f
return shamt<<20 | (rs1+8)<<15 | 5<<12 | (rs1+8)<<7 | 0x13
case 0b01: // C.SRAI = srai rs1+8, rs1+8, shamt
rs1 := (instr >> 7) & 0x7
shamt := (instr>>7)&0x20 | (instr>>2)&0x1f
return 0x20<<25 | shamt<<20 | (rs1+8)<<15 | 5<<12 | (rs1+8)<<7 | 0x13
case 0b10: // C.ANDI = andi, r+8, r+8, imm
r := (instr >> 7) & 0x7
imm := (instr>>7)&0x20 | (instr>>2)&0x1f
if instr&0x1000 != 0 {
imm |= 0xffffffc0
}
return imm<<20 | (r+8)<<15 | 7<<12 | (r+8)<<7 | 0x13
case 0b11:
funct1 := (instr >> 12) & 1
funct22 := (instr >> 5) & 0x3
rs1 := (instr >> 7) & 0x7
rs2 := (instr >> 2) & 0x7
switch funct1 {
case 0:
switch funct22 {
case 0b00: // C.SUB = sub rs1+8, rs1+8, rs2+8
return 0x20<<25 | (rs2+8)<<20 | (rs1+8)<<15 | (rs1+8)<<7 | 0x33
case 0b01: // C.XOR = xor rs1+8, rs1+8, rs2+8
return (rs2+8)<<20 | (rs1+8)<<15 | 4<<12 | (rs1+8)<<7 | 0x33
case 0b10: // C.OR = or rs1+8, rs1+8, rs2+8
return (rs2+8)<<20 | (rs1+8)<<15 | 6<<12 | (rs1+8)<<7 | 0x33
case 0b11: // C.AND = and rs1+8, rs1+8, rs2+8
return (rs2+8)<<20 | (rs1+8)<<15 | 7<<12 | (rs1+8)<<7 | 0x33
default:
panic("unreachable")
}
case 1:
switch funct22 {
case 0b00: // C.SUBW = subw r1+8, r1+8, r2+8
return 0x20<<25 | (rs2+8)<<20 | (rs1+8)<<15 | (rs1+8)<<7 | 0x3b
case 0b01: // C.ADDW = addw r1+8, r1+8, r2+8
return (rs2+8)<<20 | (rs1+8)<<15 | (rs1+8)<<7 | 0x3b
case 0b10:
panic("reserved")
case 0b11:
panic("reserved")
default:
panic("unreachable")
}
default:
panic("unreachable")
}
default:
panic("unreachable")
}
case 0b101: // C.J = jal x0, imm
offset := (instr>>1)&0x800 | (instr>>7)&0x10 | (instr>>1)&0x300 | (instr<<2)&0x400 | (instr>>1)&0x40 | (instr<<1)&0x80 | (instr>>2)&0xe | (instr<<3)&0x20
if instr&0x1000 != 0 {
offset |= 0xfffff000
}
imm := (offset>>1)&0x80000 | (offset<<8)&0x7fe00 | (offset>>3)&0x100 | (offset>>12)&0xff
return imm<<12 | 0x6f
case 0b110: // C.BEQZ = beq r+8, x0, offset
r := (instr >> 7) & 0x7
offset := (instr>>4)&0x100 | (instr>>7)&0x18 | (instr<<1)&0xc0 | (instr>>2)&0x6 | (instr<<3)&0x20
if instr&0x1000 != 0 {
offset |= 0xfffffe00
}
imm2 := (offset>>6)&0x40 | (offset>>5)&0x3f
imm1 := (offset>>0)&0x1e | (offset>>11)&0x1
return imm2<<25 | (r+8)<<20 | imm1<<7 | 0x63
case 0b111: // C.BNEZ = bne r+8, x0, offset
r := (instr >> 7) & 0x7
offset := (instr>>4)&0x100 | (instr>>7)&0x18 | (instr<<1)&0xc0 | (instr>>2)&0x6 | (instr<<3)&0x20
if instr&0x1000 != 0 {
offset |= 0xfffffe00
}
imm2 := (offset>>6)&0x40 | (offset>>5)&0x3f
imm1 := (offset>>0)&0x1e | (offset>>11)&0x1
return imm2<<25 | (r+8)<<20 | 1<<12 | imm1<<7 | 0x63
default:
panic("unreachable")
}
case 0b10:
switch funct3 {
case 0b000: // C.SLLI = slli r, r, shamt
r := (instr >> 7) & 0x1f
shamt := (instr>>7)&0x20 | (instr>>2)&0x1f
if r != 0 {
return shamt<<20 | r<<15 | 1<<12 | r<<7 | 0x13
} else {
panic("reserved")
}
case 0b001:
panic("nyi - C.FLDSP/C.LQSP")
case 0b010: // C.LWSP = lw r, offset(x2)
r := (instr >> 7) & 0x1f
offset := (instr>>7)&0x20 | (instr>>2)&0x1c | (instr<<4)&0xc0
if r != 0 {
return offset<<20 | 2<<15 | 2<<12 | r<<7 | 0x3
} else {
panic("reserved")
}
case 0b011: // C.LDSP = ld rd, offset(x2)
rd := (instr >> 7) & 0x1f
offset := (instr>>7)&0x20 | (instr>>2)&0x18 | (instr<<4)&0x1c0
if rd != 0 {
return offset<<20 | 2<<15 | 3<<12 | rd<<7 | 0x3
} else {
panic("reserved")
}
case 0b100:
rs1 := (instr >> 7) & 0b11111
rs2 := (instr >> 2) & 0b11111
if instr&0x1000 == 0 {
if rs1 == 0 {
panic("reserved")
} else {
if rs2 == 0 { // C.JR
return (rs1 << 15) | 0x67
} else { // C.MV
return (rs2 << 20) | (rs1 << 7) | 0x33
}
}
} else {
if rs2 == 0 {
if rs1 == 0 { // C.EBREAK
return 0x00100073
} else { // C.JALR
return (rs1 << 15) | (1 << 7) | 0x67
}
} else {
if rs1 == 0 {
panic("reserved")
} else { // C.ADD
return (rs2 << 20) | (rs1 << 15) | (rs1 << 7) | 0x33
}
}
}
case 0b101: // C.FSDSP = fsd rs2, offset(x2)
rs2 := (instr >> 2) & 0x1f
offset := (instr>>7)&0x38 | (instr>>1)&0x1c0
imm115 := (offset >> 5) & 0x3f
imm40 := offset & 0x1f
return imm115<<25 | rs2<<20 | 2<<15 | 3<<12 | imm40<<7 | 0x27
case 0b110: // C.SWSP = sw rs2, offset(x2)
rs2 := (instr >> 2) & 0x1f
offset := (instr>>7)&0x3c | (instr>>1)&0xc0
imm115 := (offset >> 5) & 0x3f
imm40 := offset & 0x1f
return imm115<<25 | rs2<<20 | 2<<15 | 2<<12 | imm40<<7 | 0x23
case 0b111: // C.SDSP = sd rs, offset(x2)
rs2 := (instr >> 2) & 0x1f
offset := (instr>>7)&0x38 | (instr>>1)&0x1c0
imm115 := (offset >> 5) & 0x3f
imm40 := offset & 0x1f
return imm115<<25 | rs2<<20 | 2<<15 | 3<<12 | imm40<<7 | 0x23
default:
panic("unreachable")
}
default:
panic("compressed instruction cannot be 0b11")
}
}
const (
IER_RXINT_BIT uint8 = 0x1
IER_THREINT_BIT uint8 = 0x2
IIR_THR_EMPTY uint8 = 0x2
IIR_RD_AVAILABLE uint8 = 0x4
IIR_NO_INTERRUPT uint8 = 0x7
LSR_DATA_AVAILABLE uint8 = 0x1
LSR_THR_EMPTY uint8 = 0x20
)
const (
DISK_IRQ uint32 = 1
UART_IRQ uint32 = 10
)
type Plic struct {
irq uint32
enabled uint64 // TODO: Contexts other than 1?
threshold uint32
ips [1024]uint8
priorities [1024]uint32
updateIRQ bool
lastdiskip bool
}
func NewPlic() Plic {
return Plic{}
}
func (plic *Plic) step(clock uint64, uartip, diskip bool, mip *uint64) {
// TOOD: Generalize to set of connected interrupt sources
if diskip != plic.lastdiskip { // Track edge of ip signal and trigger on raising edge only
if diskip { // Disk is interrupting
index := DISK_IRQ >> 3
plic.ips[index] |= 1 << (DISK_IRQ & 7)
plic.updateIRQ = true
}
plic.lastdiskip = diskip
}
if uartip { // Uart is interrupting
index := UART_IRQ >> 3
plic.ips[index] |= 1 << (UART_IRQ & 7)
plic.updateIRQ = true
}
if plic.updateIRQ {
diskip := (plic.ips[DISK_IRQ>>3]>>(DISK_IRQ&7))&1 == 1
diskpri := plic.priorities[DISK_IRQ]
diskenabled := (plic.enabled>>DISK_IRQ)&1 == 1
uartip := (plic.ips[UART_IRQ>>3]>>(UART_IRQ&7))&1 == 1
uartpri := plic.priorities[UART_IRQ]
uartenabled := (plic.enabled>>UART_IRQ)&1 == 1
irq := uint32(0)
pri := uint32(0)
if diskip && diskenabled && diskpri > plic.threshold && diskpri > pri {
irq = DISK_IRQ
pri = diskpri
}
if uartip && uartenabled && uartpri > plic.threshold && uartpri > pri {
irq = UART_IRQ
pri = uartpri
}
plic.irq = irq
if plic.irq != 0 {
*mip |= MIP_SEIP
}
plic.updateIRQ = false
}
}
func (plic *Plic) readuint8(addr uint64) (v uint8) {
// defer func() { fmt.Printf("plic[%x] => %x\n", addr, v) }()
if addr >= 0x0c000000 && addr <= 0x0c000fff {
panic("nyi - read from plicpriorities")
} else if addr >= 0x0c001000 && addr <= 0x0c00107f {
panic("nyi - read from plic ips")
} else if addr >= 0x0c002080 && addr <= 0x0c002087 {
return uint8(plic.enabled >> ((addr - 0x0c002080) * 8))
} else if addr >= 0x0c201000 && addr <= 0x0c201003 {
return uint8(plic.threshold >> ((addr - 0x0c201000) * 8))
} else if addr >= 0x0c201004 && addr <= 0x0c201007 {
return uint8(plic.irq >> ((addr - 0x0c201004) * 8))
} else {
fmt.Printf("warning: ignored plic[%x] => \n", addr)
return 0
}
}
func (plic *Plic) writeuint8(addr uint64, v uint8) {
// fmt.Printf("plic[%x] <= %x\n", addr, v)
if addr >= 0x0c000000 && addr <= 0x0c000fff {
offset := addr & 0b11
index := (addr - 0xc000000) >> 2
pos := offset << 3
plic.priorities[index] = plic.priorities[index]&^(0xff<<pos) | uint32(v)<<pos
plic.updateIRQ = true
} else if addr >= 0x0c002080 && addr <= 0x0c002087 {
pos := 8 * (addr & 0b111)
plic.enabled = plic.enabled & ^(0xff<<pos) | uint64(v)<<pos
if pos == 0 {
plic.updateIRQ = true
}
} else if addr >= 0x0c201000 && addr <= 0x0c201003 {
pos := 8 * (addr & 0b11)
plic.threshold = plic.threshold & ^(0xff<<pos) | uint32(v)<<pos
if pos == 0 {
plic.updateIRQ = true
}
} else if addr == 0x0c201004 {
index := v >> 3
plic.ips[index] &= ^(1 << (v & 0b111))
plic.updateIRQ = true
} else {
// fmt.Printf("warning: ignored plic[%x] <= %x\n", addr, v)
}
}
type Uart struct {
rbr uint8
thr uint8
ier uint8
iir uint8
lcr uint8
mcr uint8
lsr uint8
scr uint8
threip bool
interrupting bool
inputBuffer bytes.Buffer
}
func NewUart(screen tcell.Screen) *Uart {
uart := &Uart{
lsr: LSR_THR_EMPTY,
}
if screen != nil {
go func() {
for {
ev := screen.PollEvent()
switch ev := ev.(type) {
case *tcell.EventKey:
switch ev.Key() {
case tcell.KeyRune:
var b [4]byte
n := utf8.EncodeRune(b[:], ev.Rune())
if n == 1 {
uart.inputBuffer.WriteByte(b[0])
} else {
panic(b)
}
case tcell.KeyCtrlD:
screen.Fini()
panic("ctrl-c: exiting")
case tcell.KeyUp:
uart.inputBuffer.Write([]byte{27, 91, 65})
case tcell.KeyDown:
uart.inputBuffer.Write([]byte{27, 91, 66})
case tcell.KeyRight:
uart.inputBuffer.Write([]byte{27, 91, 67})
case tcell.KeyLeft:
uart.inputBuffer.Write([]byte{27, 91, 68})
default:
if ev.Key()>>8 != 0 {
panic(ev.Key())
}
uart.inputBuffer.WriteByte(uint8(ev.Key()))
}
}
}
}()
}
return uart
}
func (uart *Uart) step(clock uint64) {
rxip := false
if clock%0x38400 == 0 && uart.rbr == 0 {
b, err := uart.inputBuffer.ReadByte()
if err == nil {
uart.rbr = b
uart.lsr |= LSR_DATA_AVAILABLE
uart.updateIIR()
if uart.ier&IER_RXINT_BIT != 0 {
rxip = true
}
}
}
if clock%0x10 == 0 && uart.thr != 0 {
_, err := os.Stdout.Write([]byte{uart.thr})
if err != nil {
panic("unable to write byte")
}
uart.thr = 0
uart.lsr |= LSR_THR_EMPTY
uart.updateIIR()
if uart.ier&IER_THREINT_BIT != 0 {
uart.threip = true
}
}
if uart.threip || rxip {
uart.interrupting = true
uart.threip = false
} else {
uart.interrupting = false
}
}
func (uart *Uart) updateIIR() {
rxip := uart.ier&IER_RXINT_BIT != 0 && uart.rbr != 0
threip := uart.ier&IER_THREINT_BIT != 0 && uart.thr == 0
if rxip {
uart.iir = IIR_RD_AVAILABLE
} else if threip {
uart.iir = IIR_THR_EMPTY
} else {
uart.iir = IIR_NO_INTERRUPT
}
}
func (uart *Uart) readuint8(addr uint64) (v uint8) {
// defer func() { fmt.Printf("uart[%x] => %x\n", addr, v) }()
switch addr {
case 0x10000000:
if (uart.lcr >> 7) == 0 {
rbr := uart.rbr
uart.rbr = 0
uart.lsr &= ^LSR_DATA_AVAILABLE
uart.updateIIR()
return rbr
} else {
return 0
}
case 0x10000001:
if (uart.lcr >> 7) == 0 {
return uart.ier
} else {
return 0
}
case 0x10000002:
return uart.iir
case 0x10000003:
return uart.lcr
case 0x10000004:
return uart.mcr
case 0x10000005:
return uart.lsr
case 0x10000007:
return uart.scr
default:
return 0
}
}
func (uart *Uart) writeuint8(addr uint64, v uint8) {
// fmt.Printf("uart[%x] <= %x\n", addr, v)
switch addr {
case 0x10000000:
if (uart.lcr >> 7) == 0 {
uart.thr = v
uart.lsr &= ^LSR_THR_EMPTY
uart.updateIIR()
} else {
// TODO: ??
}
case 0x10000001:
if (uart.lcr >> 7) == 0 {
if uart.ier&IER_THREINT_BIT == 0 && v&IER_THREINT_BIT != 0 && uart.thr == 0 {
uart.threip = true
}
uart.ier = v
uart.updateIIR()
} else {
// TODO: ??
}
case 0x10000003:
uart.lcr = v
case 0x10000004:
uart.mcr = v
case 0x10000007:
uart.scr = v
default:
// Do nothing
}
}
func loadElf(file string, mem []byte) (uint64, error) {
f, err := elf.Open(file)
if err != nil {
return 0, err
}
defer f.Close()
for _, prog := range f.Progs {
if prog.Paddr < MEMORYBASE {
panic("ELF memory segment below 0x80000000 mapped to RAM")
}
memaddr := prog.Paddr - MEMORYBASE
n, err := prog.ReadAt(mem[memaddr:memaddr+prog.Filesz], 0)
if err != nil {
return 0, err
}
if n != int(prog.Filesz) {
return 0, fmt.Errorf("didn't read full section")
}
for i := prog.Filesz; i < prog.Memsz; i++ {
mem[memaddr+i] = 0
}
}
return f.Entry, nil
}
type Clint struct {
msip uint32
mtimecmp uint64
mtime uint64
}
func NewClint() Clint {
return Clint{}
}
func (clint *Clint) step(clock uint64, mip *uint64) {
clint.mtime++
if clint.msip&1 != 0 {
*mip |= MIP_MSIP
}
if clint.mtimecmp > 0 && clint.mtime >= clint.mtimecmp {
*mip |= MIP_MTIP
}
}
func (clint *Clint) readuint8(addr uint64) (v uint8) {
// defer func() { fmt.Printf("clint[%x] => %x\n", addr, v) }()
// fmt.Printf("warning: ignored cint[%x] =>\n", addr)
panic("nyi - read from clint")
}
func (clint *Clint) writeuint8(addr uint64, v uint8) {
// fmt.Printf("clint[%x] <= %x\n", addr, v)
switch addr {
case 0x02000000:
clint.msip = clint.msip&^0x1 | uint32(v)&1
case 0x02000001, 0x02000002, 0x02000003:
// Hardwired to zero
case 0x02004000, 0x02004001, 0x02004002, 0x02004003,
0x02004004, 0x02004005, 0x02004006, 0x02004007:
sh := (addr - 0x02004000) * 8
clint.mtimecmp = clint.mtimecmp&^(0xff<<sh) | uint64(v)<<sh
case 0x0200bff8, 0x0200bff9, 0x0200bffa, 0x0200bffb,
0x0200bffc, 0x0200bffd, 0x0200bffe, 0x0200bfff:
sh := (addr - 0x0200bff8) * 8
clint.mtime = clint.mtime&^(0xff<<sh) | uint64(v)<<sh
default:
fmt.Printf("warning: ignored clint[%x] <= %v\n", addr, v)
}
}
type VirtioBlock struct {
data []uint64
clock uint64
notifications []uint64
usedRingIndex uint16
guestpagesize uint32
deviceFeaturesSelector uint32
deviceFeatures uint64
guestFeatures uint32
guestFeaturesSel uint32
queueSel uint32
queueNum [1]uint32
queueAlign [1]uint32
queuePFN [1]uint32
queueNotify uint32
status uint32
interruptStatus uint32
cpu *CPU
}
func NewVirtioBlock(byts []uint8, cpu *CPU) VirtioBlock {
data := make([]uint64, (len(byts)+7)/8)
for i := range byts {
data[i>>3] |= uint64(byts[i]) << ((i % 8) * 8)
}
return VirtioBlock{
cpu: cpu,
data: data,
queueAlign: [1]uint32{0x1000},
}
}
func (vb *VirtioBlock) step() {
if len(vb.notifications) != 0 && vb.clock == vb.notifications[0]+500 {
vb.interruptStatus |= 0b1
vb.handleNotification()
vb.notifications = vb.notifications[1:]
}
vb.clock++
}
func (vb *VirtioBlock) handleNotification() {
baseDescAddr := uint64(vb.queuePFN[vb.queueSel]) * uint64(vb.guestpagesize)
queueSize := uint64(vb.queueNum[vb.queueSel])
baseAvailAddr := baseDescAddr + queueSize*16
align := uint64(vb.queueAlign[vb.queueSel])
baseUsedAddr := ((baseAvailAddr + 4 + queueSize*2 + align - 1) / align) * align
descIndexAddr := baseAvailAddr + 4 + (uint64(vb.usedRingIndex)%queueSize)*2
descHeadIndex := (uint64(vb.cpu.readphysical(descIndexAddr)) + uint64(vb.cpu.readphysical(descIndexAddr+1))<<8) % queueSize
var blkSector, descNum, next uint64
for {
descElemAddr := baseDescAddr + 16*next
descAddr := vb.cpu.readphysicaluint64(descElemAddr)
descLenFlagsNext := vb.cpu.readphysicaluint64(descElemAddr + 8)
descLen := uint64(uint32(descLenFlagsNext))
descFlags := uint16(descLenFlagsNext >> 32)
descNext := uint16(descLenFlagsNext >> 48)
next = uint64(descNext) % queueSize
switch descNum {
case 0:
blkSector = vb.cpu.readphysicaluint64(descAddr + 8)
case 1:
if descFlags&0x2 == 0 { // Write
for i := uint64(0); i < descLen; i++ {
v := vb.cpu.readphysical(descAddr + i)
diskAddr := blkSector*512 + i
diskIndex := diskAddr >> 3
diskPos := (diskAddr % 8) * 8
vb.data[diskIndex] = vb.data[diskIndex]&^(0xff<<diskPos) | uint64(v)<<diskPos
}
} else { // Read
for i := uint64(0); i < descLen; i++ {
diskAddr := blkSector*512 + i
diskIndex := diskAddr >> 3
diskPos := (diskAddr % 8) * 8
v := uint8(vb.data[diskIndex] >> diskPos)
vb.cpu.writephysical(descAddr+i, v)
}
}
case 2:
if descFlags&0x2 == 0 {
panic("Third descriptor should be write.")
}
if descLen != 1 {
panic("Third descriptor length should be one.")
}
vb.cpu.writephysical(descAddr, 0)
default:
}
descNum++
if descFlags&0b1 == 0 {
break
}
}
if descNum != 3 {
panic("expected 3 element descriptor chain")
}
vb.cpu.writephysical(baseUsedAddr+4+(uint64(vb.usedRingIndex)%queueSize)*8, uint8(descHeadIndex))
vb.cpu.writephysical(baseUsedAddr+5+(uint64(vb.usedRingIndex)%queueSize)*8, uint8(descHeadIndex>>8))
vb.cpu.writephysical(baseUsedAddr+6+(uint64(vb.usedRingIndex)%queueSize)*8, uint8(descHeadIndex>>16))
vb.cpu.writephysical(baseUsedAddr+7+(uint64(vb.usedRingIndex)%queueSize)*8, uint8(descHeadIndex>>24))
vb.usedRingIndex++
vb.cpu.writephysical(baseUsedAddr+2, uint8(vb.usedRingIndex))
vb.cpu.writephysical(baseUsedAddr+3, uint8(vb.usedRingIndex>>8))
}
func (vb *VirtioBlock) readuint8(addr uint64) (v uint8) {
// defer func() { fmt.Printf("virtioblock[%x] => %x\n", addr, v) }()
switch addr {
case 0x10001000, 0x10001001, 0x10001002, 0x10001003: // MagicValue
sh := (addr - 0x10001000) * 8
return uint8(uint64(0x74726976) >> sh)
case 0x10001004, 0x10001005, 0x10001006, 0x10001007: // Version
sh := (addr - 0x10001004) * 8
return uint8(uint64(0x1) >> sh)
case 0x10001008, 0x10001009, 0x1000100a, 0x1000100b: // DeviceID
sh := (addr - 0x10001008) * 8
return uint8(uint64(0x2) >> sh)
case 0x1000100c, 0x1000100d, 0x1000100e, 0x1000100f: // VendorID
sh := (addr - 0x1000100c) * 8
return uint8(uint64(0x554d4551) >> sh)
case 0x10001010, 0x10001011, 0x10001012, 0x10001013: // HostFeatures
sh := (addr - 0x10001010) * 8
return uint8((vb.deviceFeatures >> (vb.deviceFeaturesSelector * 32)) >> sh)
case 0x10001034, 0x10001035, 0x10001036, 0x10001037: // QueueNumMax
sh := (addr - 0x10001034) * 8
maxQueueSize := 0x2000
return uint8(maxQueueSize >> sh)
case 0x10001040, 0x10001041, 0x10001042, 0x10001043: // QueuePFN
sh := (addr - 0x10001040) * 8
return uint8(vb.queuePFN[vb.queueSel] >> sh)
case 0x10001050, 0x10001051, 0x10001052, 0x10001053: // QueueNotify
sh := (addr - 0x10001050) * 8
return uint8(vb.queueNotify >> sh)
case 0x10001060, 0x10001061, 0x10001062, 0x10001063: // InterruptStatus
sh := (addr - 0x10001060) * 8
return uint8(vb.interruptStatus >> sh)
case 0x10001070, 0x10001071, 0x10001072, 0x10001073: // Status
sh := (addr - 0x10001070) * 8
return uint8(vb.status >> sh)
case 0x10001100, 0x10001101, 0x10001102, 0x10001103, 0x10001104,
0x10001105, 0x10001106, 0x10001107: // Capacity
sh := (addr - 0x10001100) * 8
return uint8(uint64(0x32000) >> sh) /// 104MB??
default:
panic("nyi - read from virtio block device")
}
}
func (vb *VirtioBlock) writeuint8(addr uint64, v uint8) {
// fmt.Printf("virtioblock[%x] <= %x\n", addr, v)
switch addr {
case 0x10001014, 0x10001015, 0x10001016, 0x10001017:
sh := (addr - 0x10001014) * 8
vb.deviceFeaturesSelector = vb.deviceFeaturesSelector&^(0xff<<sh) | uint32(v)<<sh
case 0x10001020, 0x10001021, 0x10001022, 0x10001023:
sh := (addr - 0x10001020) * 8
vb.guestFeatures = vb.guestFeatures&^(0xff<<sh) | uint32(v)<<sh
case 0x10001024, 0x10001025, 0x10001026, 0x10001027:
sh := (addr - 0x10001024) * 8
vb.guestFeaturesSel = vb.guestFeaturesSel&^(0xff<<sh) | uint32(v)<<sh
case 0x10001028, 0x10001029, 0x1000102a, 0x1000102b:
sh := (addr - 0x10001028) * 8
vb.guestpagesize = vb.guestpagesize&^(0xff<<sh) | uint32(v)<<sh
case 0x10001030, 0x10001031, 0x10001032, 0x10001033:
sh := (addr - 0x10001030) * 8
vb.queueSel = vb.queueSel&^(0xff<<sh) | uint32(v)<<sh
if addr == 0x10001033 && vb.queueSel != 0 {
panic("nyi - multi queue")
}
case 0x10001038, 0x10001039, 0x1000103a, 0x1000103b:
sh := (addr - 0x10001038) * 8
vb.queueNum[vb.queueSel] = vb.queueNum[vb.queueSel]&^(0xff<<sh) | uint32(v)<<sh
case 0x1000103c, 0x1000103d, 0x1000103e, 0x1000103f:
sh := (addr - 0x1000103c) * 8
vb.queueAlign[vb.queueSel] = vb.queueAlign[vb.queueSel]&^(0xff<<sh) | uint32(v)<<sh
case 0x10001040, 0x10001041, 0x10001042, 0x10001043:
sh := (addr - 0x10001040) * 8
vb.queuePFN[vb.queueSel] = vb.queuePFN[vb.queueSel]&^(0xff<<sh) | uint32(v)<<sh
case 0x10001050, 0x10001051, 0x10001052, 0x10001053:
sh := (addr - 0x10001050) * 8
vb.queueNotify = vb.queueNotify&^(0xff<<sh) | uint32(v)<<sh
if addr == 0x10001053 {
vb.notifications = append(vb.notifications, vb.clock)
}
case 0x10001064:
if v&0b1 == 1 {
vb.interruptStatus &= ^uint32(0b1)
}
case 0x10001065, 0x10001066, 0x10001067:
// do nothing
case 0x10001070, 0x10001071, 0x10001072, 0x10001073:
sh := (addr - 0x10001070) * 8
vb.status = vb.status&^(0xff<<sh) | uint32(v)<<sh
default:
panic("nyi - write to virtio block device")
}
}
func do() error {
mem := make([]byte, 0x10000000)
entry, err := loadElf(filepath.Join("linux", "fw_payload.elf"), mem)
if err != nil {
return err
}
rootfs, err := ioutil.ReadFile(filepath.Join("linux", "rootfs.img"))
if err != nil {
return err
}
screen, err := tcell.NewScreen()
if err != nil {
return err
}
err = screen.Init()
if err != nil {
return err
}
screen.Clear()
screen.Show()
defer screen.Fini()
cpu := NewCPU(mem, entry, rootfs, screen)
cpu.run()
return nil
}
func main() {
err := do()
if err != nil {
panic(err)
}
}
| d5a89793221b20209e80f3459272cc6cc076c447 | [
"Markdown",
"Go Module",
"Go"
] | 4 | Markdown | lukehoban/rvgo | 08a98d284c20e2bc20ab769d54f7124bf6b47542 | 0144e0a89d651b781df089aec19f8b1061a3681e | |
refs/heads/master | <repo_name>biwse/biwse-node<file_sep>/src/model/Transaction.js
/**
* Biwse API V1 Reference
* This API documentation generated from OpenAPI specification.
*
* The version of the OpenAPI document: 1.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*
*/
import ApiClient from '../ApiClient';
/**
* The Transaction model module.
* @module model/Transaction
* @version 1.0.0
*/
class Transaction {
/**
* Constructs a new <code>Transaction</code>.
* @alias module:model/Transaction
*/
constructor() {
Transaction.initialize(this);
}
/**
* Initializes the fields of this object.
* This method is used by the constructors of any subclasses, in order to implement multiple inheritance (mix-ins).
* Only for internal use.
*/
static initialize(obj) {
}
/**
* Constructs a <code>Transaction</code> from a plain JavaScript object, optionally creating a new instance.
* Copies all relevant properties from <code>data</code> to <code>obj</code> if supplied or a new instance if not.
* @param {Object} data The plain JavaScript object bearing properties of interest.
* @param {module:model/Transaction} obj Optional instance to populate.
* @return {module:model/Transaction} The populated <code>Transaction</code> instance.
*/
static constructFromObject(data, obj) {
if (data) {
obj = obj || new Transaction();
if (data.hasOwnProperty('tx_hash')) {
obj['tx_hash'] = ApiClient.convertToType(data['tx_hash'], 'String');
}
}
return obj;
}
}
/**
* Sended transaction ID
* @member {String} tx_hash
*/
Transaction.prototype['tx_hash'] = undefined;
export default Transaction;
<file_sep>/src/model/InvoiceStatus.js
/**
* Biwse API V1 Reference
* This API documentation generated from OpenAPI specification.
*
* The version of the OpenAPI document: 1.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*
*/
import ApiClient from '../ApiClient';
import InvoiceStatusData from './InvoiceStatusData';
/**
* The InvoiceStatus model module.
* @module model/InvoiceStatus
* @version 1.0.0
*/
class InvoiceStatus {
/**
* Constructs a new <code>InvoiceStatus</code>.
* @alias module:model/InvoiceStatus
*/
constructor() {
InvoiceStatus.initialize(this);
}
/**
* Initializes the fields of this object.
* This method is used by the constructors of any subclasses, in order to implement multiple inheritance (mix-ins).
* Only for internal use.
*/
static initialize(obj) {
}
/**
* Constructs a <code>InvoiceStatus</code> from a plain JavaScript object, optionally creating a new instance.
* Copies all relevant properties from <code>data</code> to <code>obj</code> if supplied or a new instance if not.
* @param {Object} data The plain JavaScript object bearing properties of interest.
* @param {module:model/InvoiceStatus} obj Optional instance to populate.
* @return {module:model/InvoiceStatus} The populated <code>InvoiceStatus</code> instance.
*/
static constructFromObject(data, obj) {
if (data) {
obj = obj || new InvoiceStatus();
if (data.hasOwnProperty('msg')) {
obj['msg'] = ApiClient.convertToType(data['msg'], 'String');
}
if (data.hasOwnProperty('data')) {
obj['data'] = InvoiceStatusData.constructFromObject(data['data']);
}
}
return obj;
}
}
/**
* Invoice's amount
* @member {String} msg
*/
InvoiceStatus.prototype['msg'] = undefined;
/**
* @member {module:model/InvoiceStatusData} data
*/
InvoiceStatus.prototype['data'] = undefined;
export default InvoiceStatus;
<file_sep>/docs/InvoiceStatus.md
# biwse.InvoiceStatus
## Properties
Name | Type | Description | Notes
------------ | ------------- | ------------- | -------------
**msg** | **String** | Invoice's amount | [optional]
**data** | [**InvoiceStatusData**](InvoiceStatusData.md) | | [optional]
<file_sep>/test/api/AppApi.spec.js
/**
* Biwse API V1 Reference
* This API documentation generated from OpenAPI specification.
*
* The version of the OpenAPI document: 1.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*
*/
(function(root, factory) {
if (typeof define === 'function' && define.amd) {
// AMD.
define(['expect.js', process.cwd()+'/src/index'], factory);
} else if (typeof module === 'object' && module.exports) {
// CommonJS-like environments that support module.exports, like Node.
factory(require('expect.js'), require(process.cwd()+'/src/index'));
} else {
// Browser globals (root is window)
factory(root.expect, root.biwse);
}
}(this, function(expect, biwse) {
'use strict';
var instance;
beforeEach(function() {
instance = new biwse.AppApi();
});
var getProperty = function(object, getter, property) {
// Use getter method if present; otherwise, get the property directly.
if (typeof object[getter] === 'function')
return object[getter]();
else
return object[property];
}
var setProperty = function(object, setter, property, value) {
// Use setter method if present; otherwise, set the property directly.
if (typeof object[setter] === 'function')
object[setter](value);
else
object[property] = value;
}
describe('AppApi', function() {
describe('createAddress', function() {
it('should call createAddress successfully', function(done) {
//uncomment below and update the code to test createAddress
//instance.createAddress(function(error) {
// if (error) throw error;
//expect().to.be();
//});
done();
});
});
describe('createInvoice', function() {
it('should call createInvoice successfully', function(done) {
//uncomment below and update the code to test createInvoice
//instance.createInvoice(function(error) {
// if (error) throw error;
//expect().to.be();
//});
done();
});
});
describe('createWalletInvoice', function() {
it('should call createWalletInvoice successfully', function(done) {
//uncomment below and update the code to test createWalletInvoice
//instance.createWalletInvoice(function(error) {
// if (error) throw error;
//expect().to.be();
//});
done();
});
});
describe('getBalance', function() {
it('should call getBalance successfully', function(done) {
//uncomment below and update the code to test getBalance
//instance.getBalance(function(error) {
// if (error) throw error;
//expect().to.be();
//});
done();
});
});
describe('getInvoice', function() {
it('should call getInvoice successfully', function(done) {
//uncomment below and update the code to test getInvoice
//instance.getInvoice(function(error) {
// if (error) throw error;
//expect().to.be();
//});
done();
});
});
describe('sendOnePayment', function() {
it('should call sendOnePayment successfully', function(done) {
//uncomment below and update the code to test sendOnePayment
//instance.sendOnePayment(function(error) {
// if (error) throw error;
//expect().to.be();
//});
done();
});
});
describe('sendPayments', function() {
it('should call sendPayments successfully', function(done) {
//uncomment below and update the code to test sendPayments
//instance.sendPayments(function(error) {
// if (error) throw error;
//expect().to.be();
//});
done();
});
});
});
}));
<file_sep>/src/model/InvoiceStatusData.js
/**
* Biwse API V1 Reference
* This API documentation generated from OpenAPI specification.
*
* The version of the OpenAPI document: 1.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*
*/
import ApiClient from '../ApiClient';
/**
* The InvoiceStatusData model module.
* @module model/InvoiceStatusData
* @version 1.0.0
*/
class InvoiceStatusData {
/**
* Constructs a new <code>InvoiceStatusData</code>.
* @alias module:model/InvoiceStatusData
*/
constructor() {
InvoiceStatusData.initialize(this);
}
/**
* Initializes the fields of this object.
* This method is used by the constructors of any subclasses, in order to implement multiple inheritance (mix-ins).
* Only for internal use.
*/
static initialize(obj) {
}
/**
* Constructs a <code>InvoiceStatusData</code> from a plain JavaScript object, optionally creating a new instance.
* Copies all relevant properties from <code>data</code> to <code>obj</code> if supplied or a new instance if not.
* @param {Object} data The plain JavaScript object bearing properties of interest.
* @param {module:model/InvoiceStatusData} obj Optional instance to populate.
* @return {module:model/InvoiceStatusData} The populated <code>InvoiceStatusData</code> instance.
*/
static constructFromObject(data, obj) {
if (data) {
obj = obj || new InvoiceStatusData();
if (data.hasOwnProperty('amount')) {
obj['amount'] = ApiClient.convertToType(data['amount'], 'Number');
}
if (data.hasOwnProperty('confs')) {
obj['confs'] = ApiClient.convertToType(data['confs'], 'Number');
}
if (data.hasOwnProperty('address')) {
obj['address'] = ApiClient.convertToType(data['address'], 'String');
}
if (data.hasOwnProperty('tx_hash')) {
obj['tx_hash'] = ApiClient.convertToType(data['tx_hash'], 'String');
}
if (data.hasOwnProperty('type')) {
obj['type'] = ApiClient.convertToType(data['type'], 'Boolean');
}
if (data.hasOwnProperty('accepted')) {
obj['accepted'] = ApiClient.convertToType(data['accepted'], 'Boolean');
}
if (data.hasOwnProperty('verification_hash')) {
obj['verification_hash'] = ApiClient.convertToType(data['verification_hash'], 'String');
}
}
return obj;
}
}
/**
* Invoice's amount
* @member {Number} amount
*/
InvoiceStatusData.prototype['amount'] = undefined;
/**
* Number of transaction confirmations
* @member {Number} confs
*/
InvoiceStatusData.prototype['confs'] = undefined;
/**
* Payment receieved on address
* @member {String} address
*/
InvoiceStatusData.prototype['address'] = undefined;
/**
* Transaction hash
* @member {String} tx_hash
*/
InvoiceStatusData.prototype['tx_hash'] = undefined;
/**
* Currency type
* @member {Boolean} type
*/
InvoiceStatusData.prototype['type'] = undefined;
/**
* Payment accepted or not
* @member {Boolean} accepted
*/
InvoiceStatusData.prototype['accepted'] = undefined;
/**
* Verification hash
* @member {String} verification_hash
*/
InvoiceStatusData.prototype['verification_hash'] = undefined;
export default InvoiceStatusData;
<file_sep>/docs/AppApi.md
# biwse.AppApi
All URIs are relative to *https://api.biwse.com/v1*
Method | HTTP request | Description
------------- | ------------- | -------------
[**createAddress**](AppApi.md#createAddress) | **POST** /app/{appId}/{coin}/address | Create new address
[**createInvoice**](AppApi.md#createInvoice) | **POST** /app/{appId}/invoice | Create invoice
[**createWalletInvoice**](AppApi.md#createWalletInvoice) | **POST** /app/{appId}/{coin}/invoice | Create wallet invoice
[**getBalance**](AppApi.md#getBalance) | **GET** /app/{appId}/{coin}/balance | Retrieve wallet balance
[**getInvoice**](AppApi.md#getInvoice) | **GET** /app/{appId}/invoice/{invoiceId} | Retreive invoice info
[**sendOnePayment**](AppApi.md#sendOnePayment) | **POST** /app/{appId}/{coin}/send | Send one payment
[**sendPayments**](AppApi.md#sendPayments) | **POST** /app/{appId}/{coin}/multi_send | Send payments
## createAddress
> Address createAddress(appId, coin)
Create new address
Create new receive address for yor wallet.
### Example
```javascript
import biwse from 'biwse';
let defaultClient = biwse.ApiClient.instance;
// Configure Bearer access token for authorization: bearerAuth
let bearerAuth = defaultClient.authentications['bearerAuth'];
bearerAuth.accessToken = "YOUR ACCESS TOKEN"
let apiInstance = new biwse.AppApi();
let appId = "appId_example"; // String | The application identidier
let coin = "coin_example"; // String | The wallet identidier
apiInstance.createAddress(appId, coin).then((data) => {
console.log('API called successfully. Returned data: ' + data);
}, (error) => {
console.error(error);
});
```
### Parameters
Name | Type | Description | Notes
------------- | ------------- | ------------- | -------------
**appId** | **String**| The application identidier |
**coin** | **String**| The wallet identidier |
### Return type
[**Address**](Address.md)
### Authorization
[bearerAuth](../README.md#bearerAuth)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
## createInvoice
> AppInvoiceCreated createInvoice(appId, appInvoice)
Create invoice
Create new invoice. In response you get url of invoice page
### Example
```javascript
import biwse from 'biwse';
let defaultClient = biwse.ApiClient.instance;
// Configure Bearer access token for authorization: bearerAuth
let bearerAuth = defaultClient.authentications['bearerAuth'];
bearerAuth.accessToken = "YOUR ACCESS TOKEN"
let apiInstance = new biwse.AppApi();
let appId = "appId_example"; // String | The application identidier
let appInvoice = new biwse.AppInvoice(); // AppInvoice |
apiInstance.createInvoice(appId, appInvoice).then((data) => {
console.log('API called successfully. Returned data: ' + data);
}, (error) => {
console.error(error);
});
```
### Parameters
Name | Type | Description | Notes
------------- | ------------- | ------------- | -------------
**appId** | **String**| The application identidier |
**appInvoice** | [**AppInvoice**](AppInvoice.md)| |
### Return type
[**AppInvoiceCreated**](AppInvoiceCreated.md)
### Authorization
[bearerAuth](../README.md#bearerAuth)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
## createWalletInvoice
> WalletInvoiceCreated createWalletInvoice(appId, coin, walletInvoice)
Create wallet invoice
Create new invoice for specific wallet. In response you get url of invoice page.
### Example
```javascript
import biwse from 'biwse';
let defaultClient = biwse.ApiClient.instance;
// Configure Bearer access token for authorization: bearerAuth
let bearerAuth = defaultClient.authentications['bearerAuth'];
bearerAuth.accessToken = "YOUR ACCESS TOKEN"
let apiInstance = new biwse.AppApi();
let appId = "appId_example"; // String | The application identidier
let coin = "coin_example"; // String | The wallet identidier
let walletInvoice = new biwse.WalletInvoice(); // WalletInvoice |
apiInstance.createWalletInvoice(appId, coin, walletInvoice).then((data) => {
console.log('API called successfully. Returned data: ' + data);
}, (error) => {
console.error(error);
});
```
### Parameters
Name | Type | Description | Notes
------------- | ------------- | ------------- | -------------
**appId** | **String**| The application identidier |
**coin** | **String**| The wallet identidier |
**walletInvoice** | [**WalletInvoice**](WalletInvoice.md)| |
### Return type
[**WalletInvoiceCreated**](WalletInvoiceCreated.md)
### Authorization
[bearerAuth](../README.md#bearerAuth)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
## getBalance
> WalletBalance getBalance(appId, coin)
Retrieve wallet balance
Retrieve wallet confirmed and total balance
### Example
```javascript
import biwse from 'biwse';
let defaultClient = biwse.ApiClient.instance;
// Configure Bearer access token for authorization: bearerAuth
let bearerAuth = defaultClient.authentications['bearerAuth'];
bearerAuth.accessToken = "YOUR ACCESS TOKEN"
let apiInstance = new biwse.AppApi();
let appId = "appId_example"; // String | The application identidier
let coin = "coin_example"; // String | The wallet identidier
apiInstance.getBalance(appId, coin).then((data) => {
console.log('API called successfully. Returned data: ' + data);
}, (error) => {
console.error(error);
});
```
### Parameters
Name | Type | Description | Notes
------------- | ------------- | ------------- | -------------
**appId** | **String**| The application identidier |
**coin** | **String**| The wallet identidier |
### Return type
[**WalletBalance**](WalletBalance.md)
### Authorization
[bearerAuth](../README.md#bearerAuth)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
## getInvoice
> InvoiceStatus getInvoice(appId, invoiceId)
Retreive invoice info
Retrieve invoice with specified identifier string
### Example
```javascript
import biwse from 'biwse';
let defaultClient = biwse.ApiClient.instance;
// Configure Bearer access token for authorization: bearerAuth
let bearerAuth = defaultClient.authentications['bearerAuth'];
bearerAuth.accessToken = "YOUR ACCESS TOKEN"
let apiInstance = new biwse.AppApi();
let appId = "appId_example"; // String | The application identidier
let invoiceId = "invoiceId_example"; // String | Invoice ID
apiInstance.getInvoice(appId, invoiceId).then((data) => {
console.log('API called successfully. Returned data: ' + data);
}, (error) => {
console.error(error);
});
```
### Parameters
Name | Type | Description | Notes
------------- | ------------- | ------------- | -------------
**appId** | **String**| The application identidier |
**invoiceId** | **String**| Invoice ID |
### Return type
[**InvoiceStatus**](InvoiceStatus.md)
### Authorization
[bearerAuth](../README.md#bearerAuth)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
## sendOnePayment
> Transaction sendOnePayment(appId, coin, payment)
Send one payment
Send one payments from wallet to one recipient
### Example
```javascript
import biwse from 'biwse';
let defaultClient = biwse.ApiClient.instance;
// Configure Bearer access token for authorization: bearerAuth
let bearerAuth = defaultClient.authentications['bearerAuth'];
bearerAuth.accessToken = "YOUR ACCESS TOKEN"
let apiInstance = new biwse.AppApi();
let appId = "appId_example"; // String | The application identidier
let coin = "coin_example"; // String | The wallet identidier
let payment = new biwse.Payment(); // Payment |
apiInstance.sendOnePayment(appId, coin, payment).then((data) => {
console.log('API called successfully. Returned data: ' + data);
}, (error) => {
console.error(error);
});
```
### Parameters
Name | Type | Description | Notes
------------- | ------------- | ------------- | -------------
**appId** | **String**| The application identidier |
**coin** | **String**| The wallet identidier |
**payment** | [**Payment**](Payment.md)| |
### Return type
[**Transaction**](Transaction.md)
### Authorization
[bearerAuth](../README.md#bearerAuth)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
## sendPayments
> Transaction sendPayments(appId, coin, payments)
Send payments
Send payments from wallet to one or many recipents
### Example
```javascript
import biwse from 'biwse';
let defaultClient = biwse.ApiClient.instance;
// Configure Bearer access token for authorization: bearerAuth
let bearerAuth = defaultClient.authentications['bearerAuth'];
bearerAuth.accessToken = "YOUR ACCESS TOKEN"
let apiInstance = new biwse.AppApi();
let appId = "appId_example"; // String | The application identidier
let coin = "coin_example"; // String | The wallet identidier
let payments = new biwse.Payments(); // Payments |
apiInstance.sendPayments(appId, coin, payments).then((data) => {
console.log('API called successfully. Returned data: ' + data);
}, (error) => {
console.error(error);
});
```
### Parameters
Name | Type | Description | Notes
------------- | ------------- | ------------- | -------------
**appId** | **String**| The application identidier |
**coin** | **String**| The wallet identidier |
**payments** | [**Payments**](Payments.md)| |
### Return type
[**Transaction**](Transaction.md)
### Authorization
[bearerAuth](../README.md#bearerAuth)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
<file_sep>/docs/AppInvoice.md
# biwse.AppInvoice
## Properties
Name | Type | Description | Notes
------------ | ------------- | ------------- | -------------
**amountUsd** | **Number** | Invoice's amount |
**callbackLink** | **String** | Callback url | [optional]
**successUrl** | **String** | Redirect user after payment | [optional]
**invoiceNumber** | **String** | Your invoice internal identifier | [optional]
<file_sep>/docs/InvoiceStatusData.md
# biwse.InvoiceStatusData
## Properties
Name | Type | Description | Notes
------------ | ------------- | ------------- | -------------
**amount** | **Number** | Invoice's amount | [optional]
**confs** | **Number** | Number of transaction confirmations | [optional]
**address** | **String** | Payment receieved on address | [optional]
**txHash** | **String** | Transaction hash | [optional]
**type** | **Boolean** | Currency type | [optional]
**accepted** | **Boolean** | Payment accepted or not | [optional]
**verificationHash** | **String** | Verification hash | [optional]
<file_sep>/docs/Transaction.md
# biwse.Transaction
## Properties
Name | Type | Description | Notes
------------ | ------------- | ------------- | -------------
**txHash** | **String** | Sended transaction ID | [optional]
<file_sep>/src/api/AppApi.js
/**
* Biwse API V1 Reference
* This API documentation generated from OpenAPI specification.
*
* The version of the OpenAPI document: 1.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*
*/
import ApiClient from "../ApiClient";
import Address from '../model/Address';
import AppInvoice from '../model/AppInvoice';
import AppInvoiceCreated from '../model/AppInvoiceCreated';
import InvoiceStatus from '../model/InvoiceStatus';
import Payment from '../model/Payment';
import Payments from '../model/Payments';
import Transaction from '../model/Transaction';
import WalletBalance from '../model/WalletBalance';
import WalletInvoice from '../model/WalletInvoice';
import WalletInvoiceCreated from '../model/WalletInvoiceCreated';
/**
* App service.
* @module api/AppApi
* @version 1.0.0
*/
export default class AppApi {
/**
* Constructs a new AppApi.
* @alias module:api/AppApi
* @class
* @param {module:ApiClient} [apiClient] Optional API client implementation to use,
* default to {@link module:ApiClient#instance} if unspecified.
*/
constructor(apiClient) {
this.apiClient = apiClient || ApiClient.instance;
}
/**
* Create new address
* Create new receive address for yor wallet.
* @param {String} appId The application identidier
* @param {String} coin The wallet identidier
* @return {Promise} a {@link https://www.promisejs.org/|Promise}, with an object containing data of type {@link module:model/Address} and HTTP response
*/
createAddressWithHttpInfo(appId, coin) {
let postBody = null;
// verify the required parameter 'appId' is set
if (appId === undefined || appId === null) {
throw new Error("Missing the required parameter 'appId' when calling createAddress");
}
// verify the required parameter 'coin' is set
if (coin === undefined || coin === null) {
throw new Error("Missing the required parameter 'coin' when calling createAddress");
}
let pathParams = {
'appId': appId,
'coin': coin
};
let queryParams = {
};
let headerParams = {
};
let formParams = {
};
let authNames = ['bearerAuth'];
let contentTypes = [];
let accepts = ['application/json'];
let returnType = Address;
return this.apiClient.callApi(
'/app/{appId}/{coin}/address', 'POST',
pathParams, queryParams, headerParams, formParams, postBody,
authNames, contentTypes, accepts, returnType, null
);
}
/**
* Create new address
* Create new receive address for yor wallet.
* @param {String} appId The application identidier
* @param {String} coin The wallet identidier
* @return {Promise} a {@link https://www.promisejs.org/|Promise}, with data of type {@link module:model/Address}
*/
createAddress(appId, coin) {
return this.createAddressWithHttpInfo(appId, coin)
.then(function(response_and_data) {
return response_and_data.data;
});
}
/**
* Create invoice
* Create new invoice. In response you get url of invoice page
* @param {String} appId The application identidier
* @param {module:model/AppInvoice} appInvoice
* @return {Promise} a {@link https://www.promisejs.org/|Promise}, with an object containing data of type {@link module:model/AppInvoiceCreated} and HTTP response
*/
createInvoiceWithHttpInfo(appId, appInvoice) {
let postBody = appInvoice;
// verify the required parameter 'appId' is set
if (appId === undefined || appId === null) {
throw new Error("Missing the required parameter 'appId' when calling createInvoice");
}
// verify the required parameter 'appInvoice' is set
if (appInvoice === undefined || appInvoice === null) {
throw new Error("Missing the required parameter 'appInvoice' when calling createInvoice");
}
let pathParams = {
'appId': appId
};
let queryParams = {
};
let headerParams = {
};
let formParams = {
};
let authNames = ['bearerAuth'];
let contentTypes = ['application/json'];
let accepts = ['application/json'];
let returnType = AppInvoiceCreated;
return this.apiClient.callApi(
'/app/{appId}/invoice', 'POST',
pathParams, queryParams, headerParams, formParams, postBody,
authNames, contentTypes, accepts, returnType, null
);
}
/**
* Create invoice
* Create new invoice. In response you get url of invoice page
* @param {String} appId The application identidier
* @param {module:model/AppInvoice} appInvoice
* @return {Promise} a {@link https://www.promisejs.org/|Promise}, with data of type {@link module:model/AppInvoiceCreated}
*/
createInvoice(appId, appInvoice) {
return this.createInvoiceWithHttpInfo(appId, appInvoice)
.then(function(response_and_data) {
return response_and_data.data;
});
}
/**
* Create wallet invoice
* Create new invoice for specific wallet. In response you get url of invoice page.
* @param {String} appId The application identidier
* @param {String} coin The wallet identidier
* @param {module:model/WalletInvoice} walletInvoice
* @return {Promise} a {@link https://www.promisejs.org/|Promise}, with an object containing data of type {@link module:model/WalletInvoiceCreated} and HTTP response
*/
createWalletInvoiceWithHttpInfo(appId, coin, walletInvoice) {
let postBody = walletInvoice;
// verify the required parameter 'appId' is set
if (appId === undefined || appId === null) {
throw new Error("Missing the required parameter 'appId' when calling createWalletInvoice");
}
// verify the required parameter 'coin' is set
if (coin === undefined || coin === null) {
throw new Error("Missing the required parameter 'coin' when calling createWalletInvoice");
}
// verify the required parameter 'walletInvoice' is set
if (walletInvoice === undefined || walletInvoice === null) {
throw new Error("Missing the required parameter 'walletInvoice' when calling createWalletInvoice");
}
let pathParams = {
'appId': appId,
'coin': coin
};
let queryParams = {
};
let headerParams = {
};
let formParams = {
};
let authNames = ['bearerAuth'];
let contentTypes = ['application/json'];
let accepts = ['application/json'];
let returnType = WalletInvoiceCreated;
return this.apiClient.callApi(
'/app/{appId}/{coin}/invoice', 'POST',
pathParams, queryParams, headerParams, formParams, postBody,
authNames, contentTypes, accepts, returnType, null
);
}
/**
* Create wallet invoice
* Create new invoice for specific wallet. In response you get url of invoice page.
* @param {String} appId The application identidier
* @param {String} coin The wallet identidier
* @param {module:model/WalletInvoice} walletInvoice
* @return {Promise} a {@link https://www.promisejs.org/|Promise}, with data of type {@link module:model/WalletInvoiceCreated}
*/
createWalletInvoice(appId, coin, walletInvoice) {
return this.createWalletInvoiceWithHttpInfo(appId, coin, walletInvoice)
.then(function(response_and_data) {
return response_and_data.data;
});
}
/**
* Retrieve wallet balance
* Retrieve wallet confirmed and total balance
* @param {String} appId The application identidier
* @param {String} coin The wallet identidier
* @return {Promise} a {@link https://www.promisejs.org/|Promise}, with an object containing data of type {@link module:model/WalletBalance} and HTTP response
*/
getBalanceWithHttpInfo(appId, coin) {
let postBody = null;
// verify the required parameter 'appId' is set
if (appId === undefined || appId === null) {
throw new Error("Missing the required parameter 'appId' when calling getBalance");
}
// verify the required parameter 'coin' is set
if (coin === undefined || coin === null) {
throw new Error("Missing the required parameter 'coin' when calling getBalance");
}
let pathParams = {
'appId': appId,
'coin': coin
};
let queryParams = {
};
let headerParams = {
};
let formParams = {
};
let authNames = ['bearerAuth'];
let contentTypes = [];
let accepts = ['application/json'];
let returnType = WalletBalance;
return this.apiClient.callApi(
'/app/{appId}/{coin}/balance', 'GET',
pathParams, queryParams, headerParams, formParams, postBody,
authNames, contentTypes, accepts, returnType, null
);
}
/**
* Retrieve wallet balance
* Retrieve wallet confirmed and total balance
* @param {String} appId The application identidier
* @param {String} coin The wallet identidier
* @return {Promise} a {@link https://www.promisejs.org/|Promise}, with data of type {@link module:model/WalletBalance}
*/
getBalance(appId, coin) {
return this.getBalanceWithHttpInfo(appId, coin)
.then(function(response_and_data) {
return response_and_data.data;
});
}
/**
* Retreive invoice info
* Retrieve invoice with specified identifier string
* @param {String} appId The application identidier
* @param {String} invoiceId Invoice ID
* @return {Promise} a {@link https://www.promisejs.org/|Promise}, with an object containing data of type {@link module:model/InvoiceStatus} and HTTP response
*/
getInvoiceWithHttpInfo(appId, invoiceId) {
let postBody = null;
// verify the required parameter 'appId' is set
if (appId === undefined || appId === null) {
throw new Error("Missing the required parameter 'appId' when calling getInvoice");
}
// verify the required parameter 'invoiceId' is set
if (invoiceId === undefined || invoiceId === null) {
throw new Error("Missing the required parameter 'invoiceId' when calling getInvoice");
}
let pathParams = {
'appId': appId,
'invoiceId': invoiceId
};
let queryParams = {
};
let headerParams = {
};
let formParams = {
};
let authNames = ['bearerAuth'];
let contentTypes = [];
let accepts = ['application/json'];
let returnType = InvoiceStatus;
return this.apiClient.callApi(
'/app/{appId}/invoice/{invoiceId}', 'GET',
pathParams, queryParams, headerParams, formParams, postBody,
authNames, contentTypes, accepts, returnType, null
);
}
/**
* Retreive invoice info
* Retrieve invoice with specified identifier string
* @param {String} appId The application identidier
* @param {String} invoiceId Invoice ID
* @return {Promise} a {@link https://www.promisejs.org/|Promise}, with data of type {@link module:model/InvoiceStatus}
*/
getInvoice(appId, invoiceId) {
return this.getInvoiceWithHttpInfo(appId, invoiceId)
.then(function(response_and_data) {
return response_and_data.data;
});
}
/**
* Send one payment
* Send one payments from wallet to one recipient
* @param {String} appId The application identidier
* @param {String} coin The wallet identidier
* @param {module:model/Payment} payment
* @return {Promise} a {@link https://www.promisejs.org/|Promise}, with an object containing data of type {@link module:model/Transaction} and HTTP response
*/
sendOnePaymentWithHttpInfo(appId, coin, payment) {
let postBody = payment;
// verify the required parameter 'appId' is set
if (appId === undefined || appId === null) {
throw new Error("Missing the required parameter 'appId' when calling sendOnePayment");
}
// verify the required parameter 'coin' is set
if (coin === undefined || coin === null) {
throw new Error("Missing the required parameter 'coin' when calling sendOnePayment");
}
// verify the required parameter 'payment' is set
if (payment === undefined || payment === null) {
throw new Error("Missing the required parameter 'payment' when calling sendOnePayment");
}
let pathParams = {
'appId': appId,
'coin': coin
};
let queryParams = {
};
let headerParams = {
};
let formParams = {
};
let authNames = ['bearerAuth'];
let contentTypes = ['application/json'];
let accepts = ['application/json'];
let returnType = Transaction;
return this.apiClient.callApi(
'/app/{appId}/{coin}/send', 'POST',
pathParams, queryParams, headerParams, formParams, postBody,
authNames, contentTypes, accepts, returnType, null
);
}
/**
* Send one payment
* Send one payments from wallet to one recipient
* @param {String} appId The application identidier
* @param {String} coin The wallet identidier
* @param {module:model/Payment} payment
* @return {Promise} a {@link https://www.promisejs.org/|Promise}, with data of type {@link module:model/Transaction}
*/
sendOnePayment(appId, coin, payment) {
return this.sendOnePaymentWithHttpInfo(appId, coin, payment)
.then(function(response_and_data) {
return response_and_data.data;
});
}
/**
* Send payments
* Send payments from wallet to one or many recipents
* @param {String} appId The application identidier
* @param {String} coin The wallet identidier
* @param {module:model/Payments} payments
* @return {Promise} a {@link https://www.promisejs.org/|Promise}, with an object containing data of type {@link module:model/Transaction} and HTTP response
*/
sendPaymentsWithHttpInfo(appId, coin, payments) {
let postBody = payments;
// verify the required parameter 'appId' is set
if (appId === undefined || appId === null) {
throw new Error("Missing the required parameter 'appId' when calling sendPayments");
}
// verify the required parameter 'coin' is set
if (coin === undefined || coin === null) {
throw new Error("Missing the required parameter 'coin' when calling sendPayments");
}
// verify the required parameter 'payments' is set
if (payments === undefined || payments === null) {
throw new Error("Missing the required parameter 'payments' when calling sendPayments");
}
let pathParams = {
'appId': appId,
'coin': coin
};
let queryParams = {
};
let headerParams = {
};
let formParams = {
};
let authNames = ['bearerAuth'];
let contentTypes = ['application/json'];
let accepts = ['application/json'];
let returnType = Transaction;
return this.apiClient.callApi(
'/app/{appId}/{coin}/multi_send', 'POST',
pathParams, queryParams, headerParams, formParams, postBody,
authNames, contentTypes, accepts, returnType, null
);
}
/**
* Send payments
* Send payments from wallet to one or many recipents
* @param {String} appId The application identidier
* @param {String} coin The wallet identidier
* @param {module:model/Payments} payments
* @return {Promise} a {@link https://www.promisejs.org/|Promise}, with data of type {@link module:model/Transaction}
*/
sendPayments(appId, coin, payments) {
return this.sendPaymentsWithHttpInfo(appId, coin, payments)
.then(function(response_and_data) {
return response_and_data.data;
});
}
}
<file_sep>/docs/Payments.md
# biwse.Payments
## Properties
Name | Type | Description | Notes
------------ | ------------- | ------------- | -------------
**payments** | [**[Payment]**](Payment.md) | Payments array | [optional]
<file_sep>/docs/Payment.md
# biwse.Payment
## Properties
Name | Type | Description | Notes
------------ | ------------- | ------------- | -------------
**address** | **String** | Recipient address |
**amount** | **Number** | Amount to send. |
<file_sep>/docs/WalletBalance.md
# biwse.WalletBalance
## Properties
Name | Type | Description | Notes
------------ | ------------- | ------------- | -------------
**total** | **Number** | Total wallet balance. | [optional]
**confirmed** | **Number** | Fund in wallet available to withdrawal. | [optional]
<file_sep>/README.md
# Biwse Node.js Library
This API client generated from OpenAPI specification.
## Installation
```shell
npm install --save biwse
```
## Example
```javascript
const biwse = require('biwse');
const defaultClient = biwse.ApiClient.instance;
// Configure Bearer access token for authorization: bearerAuth
const bearerAuth = defaultClient.authentications['bearerAuth'];
bearerAuth.accessToken = "YOUR ACCESS TOKEN"
const api = new biwse.AppApi()
const appId = "YOUR APP ID"; // {String} The application identidier
const coin = "btc"; // {String} The wallet identidier
api.createAddress(appId, coin).then(function(data) {
console.log('API called successfully. Returned data: ' + data);
}, function(error) {
console.error(error);
});
```
## Documentation for API Endpoints
All URIs are relative to *https://api.biwse.com/v1*
Class | Method | HTTP request | Description
------------ | ------------- | ------------- | -------------
*biwse.AppApi* | [**createAddress**](docs/AppApi.md#createAddress) | **POST** /app/{appId}/{coin}/address | Create new address
*biwse.AppApi* | [**createInvoice**](docs/AppApi.md#createInvoice) | **POST** /app/{appId}/invoice | Create invoice
*biwse.AppApi* | [**createWalletInvoice**](docs/AppApi.md#createWalletInvoice) | **POST** /app/{appId}/{coin}/invoice | Create wallet invoice
*biwse.AppApi* | [**getBalance**](docs/AppApi.md#getBalance) | **GET** /app/{appId}/{coin}/balance | Retrieve wallet balance
*biwse.AppApi* | [**getInvoice**](docs/AppApi.md#getInvoice) | **GET** /app/{appId}/invoice/{invoiceId} | Retreive invoice info
*biwse.AppApi* | [**sendOnePayment**](docs/AppApi.md#sendOnePayment) | **POST** /app/{appId}/{coin}/send | Send one payment
*biwse.AppApi* | [**sendPayments**](docs/AppApi.md#sendPayments) | **POST** /app/{appId}/{coin}/multi_send | Send payments
<file_sep>/docs/WalletInvoice.md
# biwse.WalletInvoice
## Properties
Name | Type | Description | Notes
------------ | ------------- | ------------- | -------------
**amount** | **Number** | Invoice's amount |
**callbackLink** | **String** | Callback url | [optional]
**successUrl** | **String** | Redirect user after payment | [optional]
| 08c7618499ce079cfe83b97e8e49b6898375592f | [
"JavaScript",
"Markdown"
] | 15 | JavaScript | biwse/biwse-node | d06a05d6874c662601c075de635f1b215dcc0bac | 6b1e5427b5d57a782ed964d26a26dbf4157fd0b8 | |
refs/heads/master | <file_sep>//
// TLBundle.swift
// Pods
//
// Created by wade.hawk on 2017. 5. 9..
//
//
import UIKit
open class TLBundle {
open class func podBundleImage(named: String) -> UIImage? {
let podBundle = Bundle(for: TLBundle.self)
if let url = podBundle.url(forResource: "TLPhotoPickerController", withExtension: "bundle") {
let bundle = Bundle(url: url)
return UIImage(named: named, in: bundle, compatibleWith: nil)
}
return nil
}
open class func tr(_ table: String, _ key: String, _ args: CVarArg...) -> String {
var bundle = bundle()
if let languagePackPath = bundle.path(forResource: SharedLocaleManager.shared.locale.languageCode, ofType: "lproj"),
let languageBundle = Bundle(path: languagePackPath) {
bundle = languageBundle
}
let format = NSLocalizedString(key, tableName: table, bundle: bundle, comment: "")
return String(format: format, locale: SharedLocaleManager.shared.locale, arguments: args)
}
class func bundle() -> Bundle {
let podBundle = Bundle(for: TLBundle.self)
if let url = podBundle.url(forResource: "TLPhotoPicker", withExtension: "bundle") {
let bundle = Bundle(url: url)
return bundle ?? podBundle
}
return podBundle
}
}
public class SharedLocaleManager {
static let shared = SharedLocaleManager()
public var locale: Locale = .current
}
| 5bcf861c99d69f25d87f108752efe6cc8e48f6b2 | [
"Swift"
] | 1 | Swift | 5stralia/TLPhotoPicker | ee450f9aec013901ff2cbdf1d635c9d8dc223fb6 | 83de06cce2ca0f1d48f0f56ca5fe1a1e09baaf1b | |
refs/heads/master | <repo_name>cksharma11/bricks<file_sep>/src/paddle.js
class Paddle {
constructor(height, width, bottom, left, speed = 10) {
this.height = height;
this.width = width;
this.bottom = bottom;
this.left = left;
this.speed = speed;
}
moveLeft() {
this.left = Math.max(0, this.left - this.speed);
}
moveRight() {
this.left = Math.min(760, this.left + this.speed);
}
isWithinRange(difference) {
return difference > 0 && difference < this.width;
}
doesCollideWith(ball) {
const difference = ball.getPositionX() - this.left;
return this.isWithinRange(difference) && ball.getPositionY() < this.height;
}
manageCollisionWith(ball, velocity) {
const newVelocity = new Velocity(velocity.x, velocity.y);
if (this.doesCollideWith(ball)) newVelocity.negateY();
return newVelocity;
}
}
<file_sep>/src/brick.js
class Brick {
constructor(height, width, positionX, positionY) {
this.height = height;
this.width = width;
this.positionX = positionX;
this.positionY = positionY;
this.brickStore = [];
this.collideCount = 0;
}
increaseCollideCount() {
this.collideCount += 1;
}
storeBricks(brick) {
this.brickStore.push(brick);
}
checkCollision(differenceInPositionX, differenceInPositionY) {
const isWithinX =
differenceInPositionX >= 0 && differenceInPositionX <= 110;
const isWithinY = differenceInPositionY >= 0 && differenceInPositionY <= 20;
return isWithinX && isWithinY;
}
doesCollideWith(brick, ball) {
const differenceInPositionX = ball.positionX - brick.X;
const differenceInPositionY = brick.Y - ball.positionY;
return this.checkCollision(differenceInPositionX, differenceInPositionY);
}
manageCollisionWith(ball, velocity) {
const newVelocity = new Velocity(velocity.x, velocity.y);
const doesCollide = this.brickStore.some(brick => {
if (!this.doesCollideWith(brick, ball)) return false;
document.getElementById(brick.ID).remove();
delete this.brickStore[brick.ID];
this.increaseCollideCount();
return true;
});
if (doesCollide) newVelocity.negateY();
return newVelocity;
}
}
<file_sep>/src/velocity.js
class Velocity {
constructor(x, y) {
this.x = x;
this.y = y;
}
negateX() {
this.x *= -1;
}
negateY() {
this.y *= -1;
}
}
<file_sep>/src/game.js
class Game {
constructor(screen, paddle, ball, brick) {
this.screen = screen;
this.paddle = paddle;
this.ball = ball;
this.brick = brick;
}
score() {
return this.brick.collideCount;
}
lifesCount() {
return this.screen.lifeCount;
}
checkCollision() {
let velocity = this.paddle.manageCollisionWith(
this.ball,
this.ball.velocity
);
velocity = this.brick.manageCollisionWith(this.ball, velocity);
velocity = this.screen.manageCollisionWith(this.ball, velocity);
return velocity;
}
updateState() {
const velocity = this.checkCollision();
this.ball.setVelocity(velocity);
this.ball.move();
}
}
<file_sep>/src/screen.js
class Screen {
constructor(height, width) {
this.height = height;
this.width = width;
this.lifeCount = 3;
}
decreaseLifeCount() {
this.lifeCount -= 1;
}
doesRightOrLeftCollide(ball) {
return ball.getPositionX() == 920 || ball.getPositionX() == 0;
}
doesTopCollide(ball) {
return ball.getPositionY() == 559;
}
doesBottomCollide(ball) {
return ball.getPositionY() == 1;
}
manageCollisionWith(ball, velocity) {
const newVelocity = new Velocity(velocity.x, velocity.y);
if (this.doesTopCollide(ball)) newVelocity.negateY();
if (this.doesRightOrLeftCollide(ball)) newVelocity.negateX();
if (this.doesBottomCollide(ball)) {
newVelocity.negateY();
this.decreaseLifeCount();
}
return newVelocity;
}
}
<file_sep>/src/ball.js
class Ball {
constructor(radius, positionX, positionY, velocity) {
this.height = radius;
this.width = radius;
this.positionX = positionX;
this.positionY = positionY;
this.velocity = velocity;
}
move() {
this.positionX += this.velocity.x;
this.positionY += this.velocity.y;
}
setVelocity(velocity) {
this.velocity = velocity;
}
getVelocityX() {
return this.velocity.x;
}
getVelocityY() {
return this.velocity.y;
}
getPositionX() {
return this.positionX;
}
getPositionY() {
return this.positionY;
}
}
<file_sep>/test/game.test.js
const expect = chai.expect;
describe('Paddle', () => {
it('should subtract 10 from left position of paddle', () => {
const paddle = new Paddle(20, 100, 5, 430);
paddle.moveLeft();
expect(paddle)
.to.have.property('left')
.equals(420);
});
it('should add 10 to left position of paddle', () => {
const paddle = new Paddle(20, 100, 5, 430);
paddle.moveRight();
expect(paddle)
.to.have.property('left')
.equals(440);
});
it('should return true when difference between ball.x and paddle.x is between 200 and ball height is less then 25', () => {
const paddle = new Paddle(10, 100, 100, 100);
const ball = new Ball(10, 200, 10);
expect(paddle.doesCollideWith(ball)).to.equals(true);
});
it('should return false when difference between ball.x and paddle.x is not between 200 and ball height is less then 25', () => {
const paddle = new Paddle(10, 100, 100, 100);
const ball = new Ball(10, 100, 10);
expect(paddle.doesCollideWith(ball)).to.equals(false);
});
});
describe('Ball', () => {
const ball = new Ball(40, 10, 10);
it('should increase the velocity of ball', () => {
const velocity = { x: 10, y: 10 };
ball.move(velocity);
expect(ball)
.to.have.property('x')
.equals(20);
expect(ball)
.to.have.property('y')
.equals(20);
});
it('should return false when x is less then 920', () => {
expect(ball.doesRightCollide()).to.equals(false);
});
it('should return true when x is equals to 920', () => {
const ball = new Ball(40, 920, 10);
expect(ball.doesRightCollide()).to.equals(true);
});
});
describe('Velocity', () => {
it('should make velocity.x nagetive', () => {
const velocity = new Velocity(10, 10);
velocity.negateX();
expect(velocity.x).to.equals(-10);
});
it('should make velocity.y nagetive', () => {
const velocity = new Velocity(10, 10);
velocity.negateY();
expect(velocity.y).to.equals(-10);
});
it('should make velocity.x nagetive to positive value', () => {
const velocity = new Velocity(-10, -10);
velocity.negateX();
expect(velocity.x).to.equals(10);
});
it('should make velocity.y nagetive to positive value', () => {
const velocity = new Velocity(-10, -10);
velocity.negateY();
expect(velocity.y).to.equals(10);
});
});
<file_sep>/README.md
# Bricks
JavaScript version of Brick and Ball Game.
Play Now : https://cksharma11.github.io/bricks/
| 94ce4a2ad7838849f725f47319ae3d634beb5cf2 | [
"JavaScript",
"Markdown"
] | 8 | JavaScript | cksharma11/bricks | 4c93a93ce08a34c0b253c6004e6769c78b0e95eb | 8ff992b0bc564a004a40ba43e9ae07f3e0e25bd3 | |
refs/heads/master | <repo_name>aaliszt/CS-II-Data-Structures<file_sep>/Assembler/postfix.cpp
// <NAME>
// CS23001
// postfix.cpp
// Description: Reads in infix expressions from a file and converts them to postfix,
// then puts them in an specified output file or displays them to the screen.
#include <iostream>
#include <fstream>
#include <cstdlib>
#include "stack.hpp"
#include "string/string.hpp"
//Infix to Postfix
//Converts fully parenthesized infix expressions to postfix expressions
String infixToPostfix(String str){
std::vector<String> vec = str.split(' ');
Stack<String> stk;
String right = "";
String oper = "";
String left = "";
for(int i = 0; i < (int)vec.size(); ++i){
if(vec[i] == ")"){
right = stk.pop();
oper = stk.pop();
left = stk.pop();
stk.push(left + right + oper);
right = ""; // Reset the strings to be blank
left = "";
oper = "";
}
if(vec[i] != "(" && vec[i] != ";" && vec[i] != ")") // Do not push and left or right parenthesis onto the stack
stk.push(vec[i] + " "); // Also ignore the semicolon at the end of the expression
}
return stk.pop();
}
int main(int args, char* argv[]){
if(args != 3 && args != 2){// If there ar not two or three arguments the command line input was invalid
std::cout << "Error: Invalid input, try \"postfix input output(optional)\", exiting." << std::endl;
exit(1);
}
std::ifstream inputFile(argv[1]);
if(!inputFile.is_open()){
std::cout << "Input file did not open properly, exiting." << std::endl;
exit(1);
}
if(args == 2){// If no output file is specified output defaults to the screen
String temp = "";
String str = "";
String result = "";
while(!inputFile.eof()){
inputFile >> temp;
if(inputFile.eof()) break;
if(temp == ";"){
str = str + " ;";
std::cout << "Infix: " << str << std::endl; // Infix expression
result = infixToPostfix(str);
std::cout << "Postfix: " << result << std::endl << std::endl; // Postfix expression
str = "";
}
else // Add the entire line to the string
str = str + temp + " "; // Add spaces back in so the String can be split
}
}
else{// If there were not two arguments there must be three
std::ofstream outputFile(argv[2]);
if(!outputFile.is_open()){
std::cout << "Output file did not open properly, exiting." << std::endl;
exit(1);
}
String temp = "";
String str = "";
String result = "";
while(!inputFile.eof()){
inputFile >> temp;
if(inputFile.eof()) break;
if(temp == ";"){
str = str + " ;";
outputFile << "Infix: " << str << std::endl;
result = infixToPostfix(str);
outputFile << "Postfix: " << result << std::endl << std::endl;
str = "";
}
else
str = str + temp + " ";
}
}
inputFile.close();
return 0;
}
<file_sep>/Big Integer/bigint.hpp
/**
* <NAME>
* bigint.hpp
* CS 23001
*/
// Layout for the bigint class, provides function prototypes for
// member functions and member variables of the bigint class.
#ifndef BIGINT_HPP
#define BIGINT_HPP
#include <iostream>
static const int MAX_SIZE = 200;
class bigint{
public:
// Constructors
bigint();
bigint(int);
bigint(const char[]);
// Operators
bool operator==(const bigint&)const;
int operator[](int)const;
bigint operator+(const bigint&)const;
bigint operator*(const bigint&)const;
bigint timesDigit(int)const;
bigint times10(int)const;
friend std::ostream& operator<<(std::ostream&, const bigint&);
friend std::istream& operator>>(std::istream&, bigint&);
private:
int bigintArray[MAX_SIZE];
};
#endif
<file_sep>/String/test_find.cpp
// <NAME>
// TESTS: findstr, findchar
#include <iostream>
#include "string.hpp"
#include <cassert>
int main(){
{
// SETUP
String s("a");
bool found = false;
// TEST
found = s.findstr("a");
// VERIFY
assert(found == true);
}
{
// SETUP
String s("aa");
bool found = false;
// TEST
found = s.findstr("a");
// VERIFY
assert(found == true);
}
{
// SETUP
String s("aaaa");
bool found = false;
// TEST
found = s.findstr("aaaa");
// VERIFY
assert(found == true);
}
{
// SETUP
String s("Find somthing in this string this string pls pls.");
bool found = false;
// TEST
found = s.findstr(" pls");
// VERIFY
assert(found == true);
}
{
// SETUP
String s("I can't be what you want from me - well alright.");
bool found = false;
// TEST
found = s.findstr("I can");
// VERIFY
assert(found == true);
}
{
// SETUP
String s("aaaa");
bool found = false;
// TEST
found = s.findstr("aaaaaaaa");
// VERIFY
assert(found == false);
}
{
// SETUP
String s("Don't need em");
bool found = false;
// TEST
found = s.findstr("needs");
// VERIFY
assert(found == false);
}
{
// SETUP
String s("Fuck the fame and all the hype G I just wanna know if my father would ever like me.");
bool found = false;
// TEST
found = s.findstr("likes");
// VERIFY
assert(found == false);
}
{
// SETUP
String s("a");
bool found = false;
// TEST
found = s.findchar('a');
// VERIFY
assert(found == true);
}
{
// SETUP
String s("Plausible");
bool found = false;
// TEST
found = s.findchar('e');
// VERIFY
assert(found == true);
}
{
// SETUP
String s("LMAOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOO");
bool found = false;
// TEST
found = s.findchar('Z');
// VERIFY
assert(found == false);
}
{
// SETUP
String s("What the?!");
bool found = false;
// TEST
found = s.findchar(' ');
// VERIFY
assert(found == true);
}
{
// SETUP
String s("HA-HAAAAAAAAAAAAA");
bool found = false;
// TEST
found = s.findchar(' ');
// VERIFY
assert(found == false);
}
std::cout << "Done testing find methods." << std::endl;
}
<file_sep>/String/test_ctor_chararray.cpp
// <NAME>
// TEST: Char array constructor
#include "string.hpp"
#include <iostream>
#include <cassert>
int main(){
{
// SETUP
const char s[1] = { '\0' };
// TEST
String str(s);
// VERIFY
assert(str == '\0');
}
{
// SETUP
// TEST
String str("a");
// VERIFY
assert(str == "a");
std::cout << "a == " << str << std::endl;
}
{
// SETUP
// TEST
String str("abc");
// VERIFY
assert(str == "abc");
std::cout << "abc == " << str << std::endl;
}
{
// SETUP
// TEST
String str("abc123");
// VERIFY
assert(str == "abc123");
std::cout << "abc123 == " << str << std::endl;
}
{
// SETUP
// TEST
String str("abcdefghijklmnopqrstuvwxyz12345678910");
// VERIFY
assert(str == "abcdefghijklmnopqrstuvwxyz12345678910");
std::cout << "abcdefghijklmnopqrstuvwxyz12345678910 == " << str << std::endl;
}
{
// SETUP
// TEST
String str("I don't like Strings.");
// VERIFY
assert(str == "I don't like Strings.");
std::cout << "I dont't like Strings. == " << str << std::endl;
}
{
// SETUP
// TEST
String str("A A");
// VERIFY
assert(str == "A A");
std::cout << "A A == " << str << std::endl;
}
{
// SETUP
// TEST
String str(" ");
// VERIFY
assert(str == " ");
}
{
// SETUP
// TEST
String str("<NAME> - BACK AT IT AGAIN WITH THE WHITE VANS");
// VERIFY
assert(str == "<NAME> - BACK AT IT AGAIN WITH THE WHITE VANS");
std::cout << "<NAME> - BACK AT IT AGAIN WITH THE WHITE VANS == " << str << std::endl;
}
{
// SETUP
// TEST
String str("HAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHA");
// VERIFY
assert(str == "HAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHA");
std::cout << "HAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHA == " << std::endl << str << std::endl;
}
{
// SETUP
// TEST
String str("156Characters!!!156Characters!!!156Characters!!!156Characters!!!156Characters!!!156Characters!!!156Characters!!!156Characters!!!156Characters!!!156Character");
// VERIFY
assert(str == "156Characters!!!156Characters!!!156Characters!!!156Characters!!!156Characters!!!156Characters!!!156Characters!!!156Characters!!!156Characters!!!156Character");
}
{
// SETUP
// TEST
String str("microprogrammingmissapropriatingmarginalizationsmasculinizationsmicrocrystallineminiaturizationsmultiprogrammingnewsworthinessesnoncommunicatingnondeterministicnoninstitutionalintramolecularlyinterhemeshpericinterjectionallyintermidiatenessinterconnection");
// VERIFY
assert(str == "microprogrammingmissapropriatingmarginalizationsmasculinizationsmicrocrystallineminiaturizationsmultiprogrammingnewsworthinessesnoncommunicatingnondeterministicnoninstitutionalintramolecularlyinterhemeshpericinterjectionallyintermidiatenessinterconnection");
}
std::cout << "Done testing char array ctor." << std::endl;
}
<file_sep>/String/test_ctor_char.cpp
// <NAME>
// TESTS: Character constructor
#include "string.hpp"
#include <iostream>
#include <cassert>
int main(){
{
// SETUP
char ch = '\0';
// TESTS
String str(ch);
// VERIFY
assert(str == '\0');
}
{
// SETUP
char ch = 'a';
// TESTS
String str(ch);
// VERIFY
assert(str == 'a');
std::cout << "a == " << str << std::endl;
}
{
// SETUP
char ch = '1';
// TESTS
String str(ch);
// VERIFY
assert(str == '1');
std::cout << "1 == " << str << std::endl;
}
{
// SETUP
char ch = ' ';
// TESTS
String str(ch);
// VERIFY
assert(str == ' ');
}
{
// SETUP
char ch = '0';
// TESTS
String str(ch);
// VERIFY
assert(str == '0');
std::cout << "0 == " << str << std::endl;
}
{
// SETUP
char ch = '\t';
// TESTS
String str(ch);
// VERIFY
assert(str == '\t');
}
{
// SETUP
char ch = '\n';
// TESTS
String str(ch);
// VERIFY
assert(str == '\n');
}
std::cout << "Done testing char ctor." << std::endl;
}
<file_sep>/Big Integer/add.cpp
/**
* <NAME>
* add.cpp
* CS 23001
*/
// Reads data from files into bigints
#include <iostream>
#include <fstream>
#include <cstdlib>
#include "bigint.hpp"
int main(){
std::ifstream in("data1-1.txt");
if(!in.is_open()){
std::cerr << "Could not open data1-1.txt, exiting." << std::endl;
exit(1);
}
bigint numOne;
bigint numTwo;
bigint result;
while(!in.eof()){
in >> numOne;
if(in.eof()) break; // Stops output of random data due to whitespace before the eof
in >> numTwo;
std::cout << "Bigint one is: " << numOne << std::endl << std::endl;
std::cout << "Bigint two is: " << numTwo << std::endl;
result = numOne + numTwo;
std::cout <<"Sum of addition: " << result << std::endl << std::endl;
}
in.close();
return 0;
}
<file_sep>/Assembler/test_push.cpp
// <NAME>
// TESTS: Push & Pop
#include "string/string.hpp"
#include "stack.hpp"
#include <iostream>
int main(){
{
//SETUP
Stack<int> s;
//TEST
s.push(1);
//VERIFY
assert(!s.isEmpty());
assert(1 == s.pop());
}
{
//SETUP
Stack<int> s;
//TEST
s.push(1);
s.push(2);
s.push(3);
//VERIFY
assert(!s.isEmpty());
assert(3 == s.pop());
assert(2 == s.pop());
assert(1 == s.pop());
}
{
//SETUP
Stack<String> s;
//TEST
s.push("");
//VERIFY
assert(!s.isEmpty());
assert("" == s.pop());
}
{
//SETUP
Stack<String> s;
//TEST
s.push("a");
//VERIFY
assert(!s.isEmpty());
assert("a" == s.pop());
}
{
//SETUP
Stack<String> s;
//TEST
s.push("Stack!");
s.push(" ");
s.push("a");
s.push(" ");
s.push("am");
s.push(" ");
s.push("I");
s.push(" ");
s.push("Hi");
//VERIFY
assert(!s.isEmpty());
assert("Hi" == s.pop());
assert(" " == s.pop());
assert("I" == s.pop());
assert(" " == s.pop());
assert("am" == s.pop());
assert(" " == s.pop());
assert("a" == s.pop());
assert(" " == s.pop());
assert("Stack!" == s.pop());
}
{
//SETUP
Stack<double> d;
//TEST
d.push(1.1);
d.push(1.2);
d.push(1.3);
d.push(1.4);
d.push(1.5);
d.push(3.1415926535);
//VERIFY
assert(!d.isEmpty());
assert(3.1415926535 == d.pop());
assert(1.5 == d.pop());
assert(1.4 == d.pop());
assert(1.3 == d.pop());
assert(1.2 == d.pop());
assert(1.1 == d.pop());
}
{
//SETUP
Stack<char> s;
//TEST
s.push('a');
s.push('b');
s.push('x');
s.push('y');
//VERIFY
assert(!s.isEmpty());
assert('y' == s.pop());
assert('x' == s.pop());
assert('b' == s.pop());
assert('a' == s.pop());
}
{
//SETUP
Stack<String> s;
//TEST
s.push("");
s.pop();
s.push("");
s.push("a");
//VERIFY
assert(!s.isEmpty());
assert("a" == s.pop());
assert("" == s.pop());
}
{
//SETUP
Stack<String> s;
//TEST
s.push("a");
s.push("b");
s.push("c");
s.push("d");
s.push("e");
assert(s.pop() == "e");
assert(s.pop() == "d");
assert(s.pop() == "c");
s.push("f");
s.push("g");
assert(s.pop() == "g");
//VERIFY
assert(!s.isEmpty());
assert("f" == s.pop());
assert("b" == s.pop());
assert("a" == s.pop());
}
{
//SETUP
Stack<int> s;
//TEST
s.push(0);
//VERIFY
assert(!s.isEmpty());
assert(0 == s.pop());
}
std::cout << "Done testing push." << std::endl;
}
<file_sep>/Big Integer/test_times_single_digit.cpp
// bigint Test Program
//
// Tests: times_10, uses ==
//
#include <iostream>
#include <cassert>
#include "bigint.hpp"
//===========================================================================
int main () {
{
//------------------------------------------------------
// Setup fixture
bigint bi(0);
// Test
bi = bi.timesDigit(0);
// Verify
assert(bi == 0);
}
{
//------------------------------------------------------
// Setup fixture
bigint bi("1235134523435");
// Test
bi = bi.timesDigit(0);
// Verify
assert(bi == 0);
}
{
//------------------------------------------------------
// Setup fixture
bigint bi(2345256);
// Test
bi = bi.timesDigit(1);
// Verify
assert(bi == 2345256);
}
{
//------------------------------------------------------
// Setup fixture
bigint bi(25);
// Test
bi = bi.timesDigit(2);
// Verify
assert(bi == 50);
}
{
//------------------------------------------------------
// Setup fixture
bigint bi(36);
// Test
bi = bi.timesDigit(9);
// Verify
assert(bi == 324);
}
{
//------------------------------------------------------
// Setup fixture
bigint bi(25000);
// Test
bi = bi.timesDigit(2);
// Verify
assert(bi == 50000);
}
{
//------------------------------------------------------
// Setup fixture
bigint bi(2354123);
// Test
bi = bi.timesDigit(9);
// Verify
assert(bi == 21187107);
}
{
//------------------------------------------------------
// Setup fixture
bigint bi(9);
// Test
bi = bi.timesDigit(9);
// Verify
assert(bi == 81);
}
{
//------------------------------------------------------
// Setup fixture
bigint bi("124356798");
// Test
bi = bi.timesDigit(2);
// Verify
assert(bi == "248713596");
}
{
//------------------------------------------------------
// Setup fixture
bigint bi("999999999");
// Test
bi = bi.timesDigit(5);
// Verify
assert(bi == "4999999995");
}
{
//------------------------------------------------------
// Setup fixture
bigint bi("126372839384657");
// Test
bi = bi.timesDigit(8);
// Verify
assert(bi == "1010982715077256");
}
//Add test cases as needed.
std::cout << "Done testing bigint * single digit" << std::endl;
}
<file_sep>/Profiler/profile_report/logentry.hpp
#ifndef CS_LOGENTRY_H_
#define CS_LOGENTRY_H_
// File: logentry.hpp
// Date: 3 / 24 / 2016
// Author: <NAME>
// Description: Class definition for a log entry.
#include <iostream>
#include <vector>
#include "string.hpp"
////////////////////////////////////////////////////////////
class Date {
public:
Date(){ day = "", month = "", year = 0; }
Date(String);
String getDay()const{ return day; }
String getMonth()const{ return month; }
int getYear()const{ return year; }
private:
String day, month;
int year;
};
////////////////////////////////////////////////////////////
class Time {
public:
Time() { hour = 0, minute = 0, second = 0; }
Time(String);
int getHour()const{ return hour; }
int getMinute()const{ return minute; }
int getSecond()const{ return second; }
private:
int hour, minute, second;
};
////////////////////////////////////////////////////////////
class LogEntry {
public:
LogEntry() { host = "", date = Date(), time = Time(), request = "", status = "", number_of_bytes = 0; };
LogEntry(String);
int getBytes()const{ return number_of_bytes; }
String getHost()const{ return host; }
bool isEmpty()const { return (host == "" && request == "" && status == ""); }
friend std::ostream& operator<<(std::ostream&, const LogEntry&);
private:
String host;
Date date;
Time time;
String request;
String status;
int number_of_bytes;
};
// Free functions
std::vector<LogEntry> parse (std::istream&);
void output_all (std::ostream&, const std::vector<LogEntry> &);
void by_host (std::ostream&, const std::vector<LogEntry> &);
int byte_count (const std::vector<LogEntry>&);
int stoi (String, int);
#endif
<file_sep>/String/oldstring.cpp
// <NAME>
// CS 23001
// string.cpp
// Defines funtions from string.hpp
#include <iostream>
#include "string.hpp"
#include <cassert>
// Default Constructor
// Initializes the char array to 0
String::String(){ str[0] = '\0'; }
// Character Constructor
// Initializes the char array to a single char
String::String(char ch){ str[0] = ch; str[1] = '\0'; }
// Character Array Constructor
// Initializes the char array to another char array
String::String(const char s[]){
int i = 0;
while(s[i] != '\0'){
str[i] = s[i];
++i;
if(i >= capacity()-1)// If we reach capacity()-1 (255) then we are at the last index and need to add a null terminator
break;
}
str[i] = '\0';
}
// Output Operator
// Displays the String
std::ostream& operator<<(std::ostream& out, const String &rhs){
out << rhs.str;
return out;
}
// Input Operator
// Reads input one word at a time
std::istream& operator>>(std::istream &in, String &rhs){
char temp[STRING_CAPACITY];
in >> temp;
rhs = String(temp);
return in;
}
// Addtition Operators
// Combines two Strings
String String::operator+(const String &rhs)const{
if(length() == capacity()-1)// If this is at capacity we cannot add to it
return *this;
if(rhs.length() == capacity()-1)// If rhs is at capacity we cannot add to it
return rhs;
String result(str);
int offset = length();
int i = 0;
while(rhs.str[i] != '\0' && i < capacity()){
result.str[offset + i] = rhs.str[i]; // Starts at the end of this and adds rhs
++i;
}
result.str[offset + i] = '\0';
return result;
}
String operator+(const char lhs[], const String &rhs){ return String(lhs) + rhs; }
String operator+(const char lhs, const String &rhs){ return String(lhs) + rhs; }
// Substring
// Returns a substring between a given starting and ending index
String String::substr(int start, int end)const{
String result;
assert(0 <= start);
assert(start <= end);
assert(end < length());
for(int i = start; i <= end; ++i)
result.str[i - start] = str[i]; // Gets the substring out of string
result.str[end - start + 1] = '\0';
return result;
}
// Find String
// Looks for a substring inside a String and return true if found
bool String::findstr(const String &find)const{
if(find.length() == 1 && str[0] == find.str[0])// If find is one char and == this then just return true
return true;
char start = find.str[0];
char end = find.str[find.length()-1];
bool search = false;
bool found = false;
int startI = 0;
for(int i = 0; i < length(); ++i){
if(str[i] == start) // If find the first char we must start looking at the next chars
search = true;
if(search && str[i] != find.str[startI]){// If we are looking at the substring and the chars do not match then our search was not succesful
search = false;
startI = 0;
}
if(search && str[i] == find.str[startI]) // Increment startI if we find another char consistent with find
++startI;
if(search && str[i] == end && startI == find.length())// If we reach the last char in find then we have found the substring in this
return true;
}
return found;
}
// Find Char
// Looks for a character in a String and returns true if found
bool String::findchar(const char &find)const{
for(int i = 0; i < length(); ++i){
if(str[i] == find)
return true;
}
return false;
}
// Equality Operators
// Checks if two String's are equal
bool String::operator==(const String &rhs)const{
int i = 0;
while((str[i] != '\0') && (str[i] == rhs.str[i]))
++i;
return str[i] == rhs.str[i];
}
bool operator==(const char s[], const String &lhs){ return lhs == s; }
bool operator==(const char ch, const String &lhs){ return lhs == ch; }
// Subscript Operators
// Returns the value at index i in str
char String::operator[](int i)const{
assert(i >= 0);
assert(i <= STRING_CAPACITY);
return str[i];
}
char& String::operator[](int i){return str[i]; }
// Other Relational Operators
// Compares Strings
bool String::operator<(const String &rhs)const{
int i = 0;
while((str[i] != '\0') && (rhs.str[i] != '\0') && (str[i] == rhs.str[i]))
++i;
return str[i] < rhs.str[i];
}
bool operator<(const char s[], const String &s1){ return !(s1 < s); }
bool operator<(const char ch, const String &s1){ return !(s1 < ch); }
bool operator<=(const String &lhs, const String &rhs){ return ((lhs == rhs) || (lhs < rhs)); }
bool operator!=(const String &lhs, const String &rhs){ return !(lhs == rhs); }
bool operator>=(const String &lhs, const String &rhs){ return ((lhs == rhs) || !(lhs < rhs)); }
bool operator>(const String &lhs, const String &rhs){ return !(lhs < rhs); }
// Length
// Returns the length of the String
int String::length()const{
int L = 0;
while(str[L] != '\0')
++L;
return L;
}
// Capacity
// Returns the capacity of the String
int String::capacity()const{ return STRING_CAPACITY; }
<file_sep>/Big Integer/test_subscript.cpp
// bigint Test Program
//
// Tests: subscript, uses ==
//
#include <iostream>
#include <cassert>
#include "bigint.hpp"
//===========================================================================
int main () {
{
// Setup
bigint bi(4);
// Test
int digit = bi[0];
// Verify
assert(bi == 4);
assert(digit == 4);
}
{
// Setup
bigint bi(4000);
// Test
int digit = bi[2];
// Verify
assert(bi == 4000);
assert(digit == 0);
}
{
// Setup
bigint bi;
// Test
int digit = bi[0];
// Verify
assert(bi == 0);
assert(digit == 0);
}
{
// Setup
bigint bi("12351345324");
// Test
int digit = bi[10];
// Verify
assert(bi == "12351345324");
assert(digit == 1);
}
{
// Setup
bigint bi("1238345837");
// Test
int digit = bi[6];
// Verify
assert(bi == "1238345837"); //Wrong. Add test cases.
assert(digit == 8);
}
//Add test cases!!
std::cout << "Done testing subscript." << std::endl;
}
<file_sep>/Assembler/test_pop.cpp
// <NAME>
// TESTS: PoP & Push
#include "string/string.hpp"
#include "stack.hpp"
#include <iostream>
int main(){
{
//SETUP
Stack<int> s;
s.push(1);
//TEST
int i = s.pop();
//VERIFY
assert(i == 1);
}
{
//SETUP
Stack<int> s;
s.push(1);
s.push(2);
s.push(3);
//TEST
int i = s.pop();
int j = s.pop();
int k = s.pop();
//VERIFY
assert(i == 3);
assert(j == 2);
assert(k == 1);
}
{
//SETUP
Stack<int> s;
for(int i = 0; i < 100; ++i)
s.push(i);
//TEST
for(int i = 0; i < 100; ++i){
int num = s.pop();
assert(num == 99 - i);
}
}
{
//SETUP
Stack<String> s;
s.push("a");
//TEST
String str = s.pop();
//VERIFY
assert(str == "a");
}
{
//SETUP
Stack<String> s;
s.push("a");
s.push("b");
s.push("c");
//TEST
String str1 = s.pop();
String str2 = s.pop();
String str3 = s.pop();
//VERIFY
assert(str1 == "c");
assert(str2 == "b");
assert(str3 == "a");
}
{
//SETUP
Stack<String> s;
for(int i = 0; i < 100; ++i)
s.push("abc123");
//TEST
for(int i = 0; i < 100; ++i){
String str = s.pop();
assert(str == "abc123");
}
}
{
//SETUP
Stack<String> s;
s.push("a");
s.push("ab");
s.push("abc");
s.push("abcd");
s.push("abcde");
s.push("012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789");
//TEST
String str1 = s.pop();
String str2 = s.pop();
String str3 = s.pop();
String str4 = s.pop();
String str5 = s.pop();
String str6 = s.pop();
//VERIFY
assert(str6 == "a");
assert(str5 == "ab");
assert(str4 == "abc");
assert(str3 == "abcd");
assert(str2 == "abcde");
assert(str1 == "012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789");
}
std::cout << "Done testing pop." << std::endl;
}
<file_sep>/Assembler/assembler.cpp
// <NAME>
// CS23001
// assembler.cpp
// Description: Reads in infix expressions from a file and converts them to postfix,
// then uses that postfix to produce assembly text, finally puts them in an specified
// output file or displays them to the screen.
#include <iostream>
#include <fstream>
#include <cstdlib>
#include "stack.hpp"
#include "string/string.hpp"
//Infix to Postfix
//Converts fully parenthesized infix expressions to postfix expressions
String infixToPostfix(String str){
std::vector<String> vec = str.split(' ');
Stack<String> stk;
String right = "";
String oper = "";
String left = "";
for(int i = 0; i < (int)vec.size(); ++i){ // Loop through every token
if(vec[i] == ")"){
right = stk.pop();
oper = stk.pop();
left = stk.pop();
stk.push(left + right + oper);
right = ""; // Reset the strings to be blank
left = "";
oper = "";
}
if(vec[i] != "(" && vec[i] != ";" && vec[i] != ")") // Do not push and left or right parenthesis onto the stack
stk.push(vec[i] + " "); // Also ignore the semicolon at the end of the expression
}
return stk.pop();
}
//Evaluate
//Outputs assembly to an ofstream
String evaluate(String L, String t, String R, int num, std::ostream& out){
char ch = num + '0'; // Converts int to a char
String result = "TMP" + String(ch); // Result is TMPn
out << " LD " << L << std::endl;
if(t == "+") // If token was add "+"
out << " AD " << R << std::endl;
else if(t == "-") // If token was subtract "-"
out << " SB " << R << std::endl;
else if(t == "*") // If token was multiply "*"
out << " ML " << R << std::endl;
else // If token was divide "/"
out << " DV " << R << std::endl;
out << " ST " << result << std::endl;
return result;
}
//Postfix to Assembly
//Converts Postfix expressions to assembly
String postfixToAssembly(String str, std::ostream& out){
std::vector<String> vec = str.split(' ');
Stack<String> stk;
String token = "";
String right = "";
String left = "";
int tempNum = 1;
for(int i = 0; i < (int)vec.size(); ++i){
token = vec[i];
if(token != "+" && token != "-" && token != "*" && token != "/")
stk.push(token);
else{
right = stk.pop();
left = stk.pop();
stk.push(evaluate(left, token, right, tempNum, out));
++tempNum; // Increment tempNum to keep tract of new temporary value
right = "";
left = "";
}
}
return stk.pop();
}
int main(int args, char* argv[]){
if(args != 3 && args != 2){// If there ar not two or three arguments the command line input was invalid
std::cout << "Error: Invalid input, try \"postfix input output(optional)\", exiting." << std::endl;
exit(1);
}
std::ifstream inputFile(argv[1]);
if(!inputFile.is_open()){
std::cout << "Input file did not open properly, exiting." << std::endl;
exit(1);
}
if(args == 2){// If no output file is specified output defaults to the screen
String temp = "";
String str = "";
String result = "";
while(!inputFile.eof()){
inputFile >> temp;
if(inputFile.eof()) break;
if(temp == ";"){
str = str + " ;";
std::cout << "Infix: " << str << std::endl; // Infix expression
result = infixToPostfix(str);
std::cout << "Postfix: " << result << std::endl << std::endl; // Postfix expression
postfixToAssembly(result, std::cout); // Assembly
str = "";
}
else // Add the entire line to the string
str = str + temp + " "; // Add spaces back in so the String can be split
}
}
else{// If there were not two arguments there must be three
std::ofstream outputFile(argv[2]);
if(!outputFile.is_open()){
std::cout << "Output file did not open properly, exiting." << std::endl;
exit(1);
}
String temp = "";
String str = "";
String result = "";
while(!inputFile.eof()){
inputFile >> temp;
if(inputFile.eof()) break;
if(temp == ";"){
str = str + " ;";
outputFile << "Infix: " << str << std::endl;
result = infixToPostfix(str);
outputFile << "Postfix: " << result << std::endl << std::endl;
postfixToAssembly(result, outputFile);
str = "";
}
else
str = str + temp + " ";
}
}
inputFile.close();
return 0;
}
<file_sep>/String/oldstring.hpp
// <NAME>
// CS 23001
// string.hpp
// Interface definition of String class
#ifndef STRING_HPP
#define STRING_HPP
#include <iostream>
const int STRING_CAPACITY = 256;
class String {
public:
String ();
String (char);
String (const char[]);
String substr (int, int) const;
bool findstr (const String&) const;
bool findchar (const char&) const;
char& operator[] (int);
char operator[] (int) const;
int capacity () const;
int length () const;
bool operator== (const String&) const;
bool operator< (const String&) const;
String operator+ (const String&) const;
friend std::istream& operator>>(std::istream&, String&);
friend std::ostream& operator<<(std::ostream&, const String&);
private:
char str[STRING_CAPACITY];
};
String operator+ (const char[], const String&);
String operator+ (char, const String&);
bool operator== (const char[], const String&);
bool operator== (char, const String&);
bool operator< (const char[], const String&);
bool operator< (char, const String&);
bool operator<= (const String&, const String&);
bool operator!= (const String&, const String&);
bool operator>= (const String&, const String&);
bool operator> (const String&, const String&);
#endif
<file_sep>/String/test_substring.cpp
// <NAME>
// TESTS: Substring
#include "string.hpp"
#include <iostream>
#include <cassert>
int main(){
{
// SETUP
String s("a");
String sub;
// TESTS
sub = s.substr(0,0);
// VERIFY
assert(sub == "a");
}
{
// SETUP
String s("abc");
String sub;
// TESTS
sub = s.substr(0,2);
// VERIFY
assert(sub == "abc");
}
{
// SETUP
String s("abcdefghijklmnop");
String sub;
// TESTS
sub = s.substr(3,6);
// VERIFY
assert(sub == "defg");
}
{
// SETUP
String s("Cat Bat Mat Rat Vat Fat Dat Pat Sat Nat");
String sub;
// TESTS
sub = s.substr(24, 34);
// VERIFY
assert(sub == "Dat Pat Sat");
}
{
// SETUP
String s("BOOOOOOOOOOOOOOOOM");
String sub;
// TESTS
sub = s.substr(17, 17);
// VERIFY
assert(sub == "M");
}
std::cout << "Done testing substring." << std::endl;
}
<file_sep>/String/test_input.cpp
// <NAME>
// TESTS: Input operator
#include "string.hpp"
#include <iostream>
#include <fstream>
#include <cstdlib>
#include <cassert>
int main(){
std::ifstream in("input.txt");
if(!in.is_open()){
std::cerr << "Could not open input.txt, exiting." << std::endl;
exit(1);
}
String s1;
if(!in.eof())
{
in >> s1;
assert(s1 == "This");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "is");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "a");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "text");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "file");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "that");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "tests");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "the");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "input");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "method");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "of");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "string.hpp");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "and");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "checks");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "if");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "it");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "reads");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "in");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "one");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "word");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "at");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "a");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "time.");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "It");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "should");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "skip");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "all");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "spaces");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "and");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "read");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "in");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "each");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "word.");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "Here");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "is");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "a");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "316");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "character");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "string");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "with");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "no");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "spaces:");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789EndofLongString.");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "Here");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "is");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "a");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "string");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "that");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "is");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "703");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "characters:");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "0123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789END");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "Test");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "TEST");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "Test...");
}
if(!in.eof())
{
in >> s1;
assert(s1 == "ENDOFFILE.");
}
std::cout << "Done testing input." << std::endl;
in.close();
return 0;
}
<file_sep>/String/logentry.cpp
// File: logentry.cpp
// Date: 3 / 24 / 2016
// Author: <NAME>
// Description: Class implementation for a log entry.
#include <iostream>
#include <vector>
#include <cassert>
#include "string.hpp"
#include "logentry.hpp"
//////////////////////////////////////////////////////////
// REQUIRES: str contains an int, and size = length of str
// ENSURES: the int value in str is returned as an int
int stoi(String str, int size){
int val = 0;
int temp = 0;
for(int i = 0; i < size; ++i){ // While i < str.length() convert the characters in str to ints
temp = int(str[i]) - int('0'); // Converts individual character to int
val = val * 10; // Add another digit when val has more than one digit
val += temp; // Add the most recent int to the end of val
}
return val;
}
//////////////////////////////////////////////////////////
// REQUIRES: String s contains a valid date
// ENSURES: day, month, and year are initialized to the values in s
Date::Date(String s) {
std::vector<String> vec = s.split(':');
String temp = vec[0]; // Front of vec contains date
std::vector<String> vec2 = temp.split('['); // Remove excess [
String date = vec2[0]; // Now we have the date with some '/' characters
std::vector<String> vec3 = date.split('/'); // Split at each /
day = vec3[0]; // Then put each member of vec3 with the proper variable
month = vec3[1];
year = stoi(vec3[2], vec3[2].length());
}
//////////////////////////////////////////////////////////
// REQUIRES: String s contains a valid time
// ENSURES: hour, minute, and second are initialized to the values in s
Time::Time(String s) {
std::vector<String> vec = s.split(':');
hour = stoi(vec[1], vec[1].length()); // Back of vec has time, convert each String to an int
minute = stoi(vec[2], vec[2].length()); // and assign the to the proper variables
second = stoi(vec[3], vec[3].length());
}
//////////////////////////////////////////////////////////
// REQUIRES:
// ENSURES: All LogEntry member variables are initialized to the values in s, or LogEntry is empty(if s is invalid)
LogEntry::LogEntry(String s) {
std::vector<String> vec = s.split(' ');
if(vec.size() != 10){ // If size != 10 the logEntry is invalid
host = ""; // Set all members to their default values
date = Date();
time = Time();
request = "";
status = "";
number_of_bytes = 0;
}
else{
host = vec[0];
date = Date(vec[3]); // vec[3] contains both time and date
time = Time(vec[3]); // send each to their String ctor to be spilt an initialized
String front = vec[5]; // vec[5] == Front of request
std::vector<String> f = front.split('"'); // Remove the quotation mark from the front of the request
request = request + f[0] + " " + vec[6]; // Store the request with proper spacing
String back = vec[7]; // vec[7] == End of request
std::vector<String> b = back.split('"'); // Remove the quotation mark from the end of the request
request = request + " " + b[0]; // Store the request with proper spacing
status = vec[8]; // vec[8] contains status number
if(vec[9] == '-') // '-' represents 0 bytes
number_of_bytes = 0;
else
number_of_bytes = stoi(vec[9], vec[9].length());
}
}
//////////////////////////////////////////////////////////
// REQUIRES: LogEntry is valid
// ENSURES: LogEntry is put into out with the same format as the input file
std::ostream& operator<<(std::ostream& out, const LogEntry& log){
if(log.isEmpty())//If log is empty then it was not valid(output nothing)
return out;
// Writes LogEntry to out with the same format as the input files
out << log.host;
out << " - - ";
out << "[" << log.date.getDay() << "/" << log.date.getMonth() << "/" << log.date.getYear();
out << ":" << log.time.getHour() << ":" << log.time.getMinute() << ":" << log.time.getSecond() << " ";
out << "-0400] \"" << log.request << "\" " << log.status << " " << log.number_of_bytes;
out << std::endl;
return out;
}
//////////////////////////////////////////////////////////
// REQUIRES:
// ENSURES: returns a vector of LogEntrys from in
std::vector<LogEntry> parse(std::istream& in) {
std::vector<LogEntry> result;
String line;
char ch;
LogEntry log;
while(!in.eof()){ // Read in all LogEntrys
in.get(ch);
if(in.eof()) break;
if(ch == '\n'){// If we are starting a new line then we must add the previous line to the vector
log = LogEntry(line);
result.push_back(log);
line = "";
}
else
line = line + ch;
}
return result;
}
//////////////////////////////////////////////////////////
// REQUIRES:
// ENSURES: All LogEntrys in vec are output(one per line)
void output_all(std::ostream& out, const std::vector<LogEntry> &vec) {
for(int i = 0; i < (int)vec.size(); ++i)
out << vec[i];
}
//////////////////////////////////////////////////////////
// REQUIRES:
// ENSURES: All hosts of LogEntrys in vec are output(one per line)
void by_host(std::ostream& out, const std::vector<LogEntry> &vec) {
for(int i = 0; i < (int)vec.size(); ++i){
if(vec[i].getHost() != "") // Ignore invalid LogEntries
out << vec[i].getHost() << std::endl;
}
}
//////////////////////////////////////////////////////////
// REQUIRES:
// ENSURES: count = total number of bytes of all LogEntrys in vec
int byte_count(const std::vector<LogEntry> &vec) {
int count = 0;
for(int i = 0; i < (int)vec.size(); ++i)
count = count + vec[i].getBytes();
return count;
}
<file_sep>/Profiler/ASTree.cpp
/*
* ASTree.cpp
* Abstract Syntax Tree
*
* Created by <NAME>
* Copyright 2016 Kent State University. All rights reserved.
*
* Modified by: <NAME>
*
*/
#include "ASTree.hpp"
/////////////////////////////////////////////////////////////////////
// Copy constructor for srcML
//
srcML::srcML(const srcML& actual) {
tree = new AST(*(actual.tree));
}
/////////////////////////////////////////////////////////////////////
// Constant time swap for srcML
//
void srcML::swap(srcML& b) {
std::string t_header = header;
header = b.header;
b.header = t_header;
AST *temp = tree;
tree = b.tree;
b.tree = temp;
}
/////////////////////////////////////////////////////////////////////
// Assignment for srcML
//
srcML& srcML::operator=(srcML rhs) {
swap(rhs);
return *this;
}
/////////////////////////////////////////////////////////////////////
// Reads in and constructs a srcML object.
//
std::istream& operator>>(std::istream& in, srcML& src){
char ch;
if (!in.eof()) in >> ch;
src.header = readUntil(in, '>');
if (!in.eof()) in >> ch;
if (src.tree) delete src.tree;
src.tree = new AST(category, readUntil(in, '>'));
src.tree->read(in);
return in;
}
/////////////////////////////////////////////////////////////////////
// Prints out a srcML object
//
std::ostream& operator<<(std::ostream& out, const srcML& src){
src.tree->print(out);
return out;
}
/////////////////////////////////////////////////////////////////////
// Adds in the includes and profile variables
//
void srcML::mainHeader(std::vector<std::string>& profileNames) {
tree->mainHeader(profileNames);
}
/////////////////////////////////////////////////////////////////////
// Adds in the includes and profile variables
//
void srcML::fileHeader(const std::string& profileName) {
tree->fileHeader(profileName);
}
/////////////////////////////////////////////////////////////////////
// Adds in the report to the main.
//
void srcML::mainReport(std::vector<std::string>& profileNames) {
tree->mainReport(profileNames);
}
/////////////////////////////////////////////////////////////////////
// Inserts a filename.count() into each function body.
//
void srcML::funcCount(const std::string& profilename) {
tree->funcCount(profilename);
}
/////////////////////////////////////////////////////////////////////
// Inserts a filename.count() for each statement.
//
void srcML::lineCount(const std::string& profilename) {
tree->lineCount(profilename);
}
/////////////////////////////////////////////////////////////////////
// Constructs a category, token, or whitespace node for the tree.
//
AST::AST(nodes t, const std::string& s) {
nodeType = t;
switch (nodeType) {
case category:
tag = s;
break;
case token:
text = unEscape(s);
break;
case whitespace:
text = s;
break;
}
}
/////////////////////////////////////////////////////////////////////
/// Destructor for AST
///
AST::~AST(){
for(std::list<AST*>::iterator i = child.begin(); i != child.end(); ++i)
delete *i;
}
/////////////////////////////////////////////////////////////////////
// Copy Constructor for AST
//
AST::AST(const AST& actual) {
text = actual.text;
tag = actual.tag;
closeTag = actual.closeTag;
nodeType = actual.nodeType;
for (std::list<AST*>::const_iterator i = actual.child.begin(); i != actual.child.end(); ++i)
child.push_back(new AST(*(*i)));
}
/////////////////////////////////////////////////////////////////////
// Constant time swap for AST
//
void AST::swap(AST& rhs) {
std::string tempTxt = rhs.text;
rhs.text = text;
text = tempTxt;
std::string tempTag = rhs.tag;
rhs.tag = tag;
tag = tempTag;
std::string tempC = rhs.closeTag;
rhs.closeTag = closeTag;
closeTag = tempC;
nodes tempN = rhs.nodeType;
rhs.nodeType = nodeType;
nodeType = tempN;
std::list<AST*> temp = child;
child = rhs.child;
rhs.child = temp;
}
/////////////////////////////////////////////////////////////////////
// Assignment for AST
//
AST& AST::operator=(AST rhs) {
swap(rhs);
return *this;
}
/////////////////////////////////////////////////////////////////////
// Returns a pointer to child[i] where (child[i]->tag == tagName)
//
// IMPORTANT for part 3
AST* AST::getChild(std::string tagName) {
std::list<AST*>::iterator ptr = child.begin();
while (((*ptr)->tag != tagName) && (ptr != child.end())) {
++ptr;
}
return *ptr;
}
/////////////////////////////////////////////////////////////////////
// Returns the full name of a <name> node.
//
// IMPORTANT for part 3
//
std::string AST::getName() const {
std::string result;
if (child.front()->tag != "name") {
result = child.front()->text; //A simple name (e.g., main)
} else { //A complex name (e.g., stack::push).
result = child.front()->child.front()->text;
result += "::";
result += child.back()->child.front()->text;
}
return result;
}
/////////////////////////////////////////////////////////////////////
// Adds in the includes and profile variables in a main file.
//
void AST::mainHeader(std::vector<std::string>& profileNames) {
for(std::list<AST*>::iterator it = child.begin(); it != child.end(); ++it){
if((*it)->tag == "function"){// When we find the first function tag stop and insert code
child.insert(it, new AST(token, "\n"));
child.insert(it, new AST(token, "#include \"profile.hpp\"\n")); // Add include derective
for(int i = 0; i < (int)profileNames.size(); ++i){// Go through filenames and insert code to create profile objects
std::string filename = profileNames[i];
for(int j = 0; j < (int)filename.size(); ++j){// Replace _ with . in filenames
if(filename[j] == '_' && filename[j+1] == 'c')
filename[j] = '.';
}
child.insert(it, new AST(token, "profile " + profileNames[i] + "(\"" + filename + "\");" + "\n"));
}
child.insert(it, new AST(token, "\n"));
break;// Leave the loop after we insert the code(stops multiple insertion)
}
}
}
/////////////////////////////////////////////////////////////////////
// Adds in the includes and profile variables for non-main files
//
void AST::fileHeader(const std::string& profileName) {
for(std::list<AST*>::iterator it = child.begin(); it != child.end(); ++it){
if((*it)->tag == "function"){
child.insert(it, new AST(token, "\n"));
child.insert(it, new AST(token, "#include \"profile.hpp\"\n")); // Add include derective
child.insert(it, new AST(token, "extern profile " + profileName + ";" + "\n"));// Add extern statement
child.insert(it, new AST(token, "\n"));
break;//Leave loop after inserting code
}
}
}
/////////////////////////////////////////////////////////////////////
// Adds in the report to the main.
// Assumes only one return at end of main body.
//
void AST::mainReport(std::vector<std::string>& profileNames) {
for(std::list<AST*>::iterator i = child.begin(); i != child.end(); ++i){
if((*i)->tag == "function" && (*i)->getChild("name")->getName() == "main"){ // If we reach a function named main
AST* main = (*i)->getChild("block");
std::list<AST*>::iterator it = main->getChild("return")->child.begin(); // Put an iterator at the front of the return block in main
for(int n = 0; n < (int)profileNames.size(); ++n)
child.insert(it, new AST(token, "std::cout << " + profileNames[n] + " << std::endl;\n "));
}
}
}
/////////////////////////////////////////////////////////////////////
// Adds in a line to count the number of times each function is executed.
// Assumes no nested functions.
//
void AST::funcCount(const std::string& profileName) {
for(std::list<AST*>::iterator i = child.begin(); i != child.end(); ++i){
if((*i)->tag == "function" || (*i)->tag == "constructor" || (*i)->tag == "destructor"){ // If we reach a function, constructor, or destructor we must add code
std::string funcName = (*i)->getChild("name")->getName(); // Get function name
AST* body = (*i)->getChild("block"); // Get a pointer to the main block of the function
std::list<AST*>::iterator it = body->child.begin(); // Put an iterator at the start of the main block
child.insert(++it, new AST(token, " " + profileName + ".count(__LINE__, \"" + funcName + "\");"));
}
}
}
/////////////////////////////////////////////////////////////////////
// Adds in a line to count the number of times each statement is executed.
// No breaks, returns, throw etc.
// Assumes all construts (for, while, if) have { }.
//
void AST::lineCount(const std::string& profileName) {
// Recursively check for expr_stmt
for(std::list<AST*>::iterator i = child.begin(); i != child.end(); ++i){
if((*i)->tag == "expr_stmt"){ // When we reach an expression statment we must insert
std::list<AST*>::iterator it = i; // Copy iterator i to prevent double insertion
child.insert(++it, new AST(token, " " + profileName + ".count(__LINE__);"));
}
else // If we are not at an expression statment continue recursing
(*i)->lineCount(profileName);
}
}
/////////////////////////////////////////////////////////////////////
// Read in and construct AST
// REQUIRES: '>' was previous charater read
// && this == new AST(category, "TagName")
//
//
std::istream& AST::read(std::istream& in) {
AST *subtree;
std::string temp, Lws, Rws;
char ch;
if (!in.eof()) in.get(ch);
while (!in.eof()) {
if (ch == '<') { //Found a tag
temp = readUntil(in, '>');
if (temp[0] == '/') {
closeTag = temp;
break; //Found close tag, stop recursion
}
subtree = new AST(category, temp); //New subtree
subtree->read(in); //Read it in
in.get(ch);
child.push_back(subtree); //Add it to child
} else { //Found a token
temp = std::string(1, ch) + readUntil(in, '<'); //Read it in.
std::vector<std::string> tokenList = tokenize(temp);
for (std::vector<std::string>::const_iterator i=tokenList.begin();
i != tokenList.end(); ++i) {
if (isspace((*i)[0])) {
subtree = new AST(whitespace, *i);
} else {
subtree = new AST(token, *i);
}
child.push_back(subtree);
}
ch = '<';
}
}
return in;
}
/////////////////////////////////////////////////////////////////////
// Print an AST
// REQUIRES: indent >= 0
//
std::ostream& AST::print(std::ostream& out) const {
for (std::list<AST*>::const_iterator i = child.begin();
i != child.end(); ++i) {
switch ((*i)->nodeType) {
case category:
(*i)->print(out);
break;
case token:
out << (*i)->text;
break;
case whitespace:
out << (*i)->text;
break;
}
}
return out;
}
/////////////////////////////////////////////////////////////////////
// Utilities
//
// IMPORTANT for part 3
bool isStopTag(std::string tag) {
if (tag == "decl_stmt" ) return true;
if (tag == "argument_list" ) return true;
if (tag == "init" ) return true;
if (tag == "condition" ) return true;
if (tag == "cpp:include" ) return true;
if (tag == "macro" ) return true;
if (tag == "comment type\"block\"") return true;
if (tag == "comment type\"line\"" ) return true;
return false;
}
//For reading
/////////////////////////////////////////////////////////////////////
// Reads until a key is encountered. Does not include ch.
// REQUIRES: in.open()
// ENSURES: RetVal[i] != key for all i.
//
std::string readUntil(std::istream& in, char key) {
std::string result;
char ch;
in.get(ch);
while (!in.eof() && (ch != key)) {
result += ch;
in.get(ch);
}
return result;
}
/////////////////////////////////////////////////////////////////////
// Converts escaped XML charaters back to charater form
// REQUIRES: s == "<"
// ENSURES: RetVal == "<"
//
std::string unEscape(std::string s) {
std::size_t pos = 0;
while ((pos = s.find(">")) != s.npos) { s.replace(pos, 4, ">");}
while ((pos = s.find("<")) != s.npos) { s.replace(pos, 4, "<");}
while ((pos = s.find("&")) != s.npos) { s.replace(pos, 5, "&");}
return s;
}
/////////////////////////////////////////////////////////////////////
// Given: s == " a + c "
// RetVal == {" ", "a", " ", "+", "c", " "}
//
std::vector<std::string> tokenize(const std::string& s) {
std::vector<std::string> result;
std::string temp = "";
unsigned i = 0;
while (i < s.length()) {
while (isspace(s[i]) && (i < s.length())) {
temp.push_back(s[i]);
++i;
}
if (temp != "") {
result.push_back(temp);
temp = "";
}
while (!isspace(s[i]) && (i < s.length())) {
temp.push_back(s[i]);
++i;
}
if (temp != "") {
result.push_back(temp);
temp = "";
}
}
return result;
}
<file_sep>/String/test_resize.cpp
// <NAME>
// TESTS: resetCapacity
#include <iostream>
#include <cassert>
#include "string.hpp"
int main(){
{
// SETUP
String s;
// TEST
s.resetCapacity(0);
// VERIFY
assert(0 == s.capacity());
}
{
// SETUP
String s("abc");
// TEST
s.resetCapacity(1);
// VERIFY
assert(1 == s.capacity());
assert(s == "");
}
{
// SETUP
String s("abc");
// TEST
s.resetCapacity(2);
// VERIFY
assert(2 == s.capacity());
assert(s == "a");
}
{
// SETUP
String s;
// TEST
s.resetCapacity(300);
// VERIFY
assert(300 == s.capacity());
}
{
// SETUP
String s("a");
// TEST
s.resetCapacity(1);
// VERIFY
assert(1 == s.capacity());
assert(s == "");
}
{
// SETUP
String s("abc");
// TEST
s.resetCapacity(300);
// VERIFY
assert(300 == s.capacity());
assert(s == "abc");
}
{
// SETUP
String s("abc");
// TEST
s.resetCapacity(2);
// VERIFY
assert(2 == s.capacity());
assert(s == "a");
}
{
// SETUP
String s("abc");
// TEST
s.resetCapacity(1);
// VERIFY
assert(1 == s.capacity());
assert(s == "");
}
{
// SETUP
String s("AYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYY LMAOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOO");
// TEST
s.resetCapacity(4);
// VERIFY
assert(4 == s.capacity());
assert(s == "AYY");
}
{
// SETUP
String s("Gotem");
// TEST
s.resetCapacity(256);
// VERIFY
assert(256 == s.capacity());
assert(s == "Gotem");
}
{
// SETUP
String s(" ");
// TEST
s.resetCapacity(5);
// VERIFY
assert(5 == s.capacity());
assert(s == " ");
}
{
// SETUP
String s("HAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHA123456");
// TEST
s.resetCapacity(256);
// VERIFY
assert(s == "HAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHA12345");
assert(256 == s.capacity());
}
{
// SETUP
String s("HAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHA12345");
// TEST
s.resetCapacity(256);
// VERIFY
assert(256 == s.capacity());
assert(s == "HAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHA12345");
}
{
// SETUP
String s("HAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHA123456789");
// TEST
s.resetCapacity(260);
// VERIFY
assert(260 == s.capacity());
assert(s == "HAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHA123456789");
}
{
// SETUP
String s("OOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOO");
// TEST
s.resetCapacity(0);
// VERIFY
assert(0 == s.capacity());
}
std::cout << "Done testing resetCapacity." << std::endl;
}
<file_sep>/String/test_length.cpp
// <NAME>
// TESTS: Length method
#include "string.hpp"
#include <iostream>
#include <cassert>
int main(){
{
// TEST
String s;
// VERIFY
assert(0 == s.length());
}
{
// TEST
String s("5");
// VERIFY
assert(1 == s.length());
}
{
// TEST
String s("abcde");
// VERIFY
assert(5 == s.length());
}
{
// TEST
String s(" ");
// VERIFY
assert(10 == s.length());
}
{
// TEST
String s("abcdefghijklmnopqrstuvwxyz1234567890");
// VERIFY
assert(36 == s.length());
}
{
// TEST
String s;
// VERIFY
assert(256 == s.capacity());
}
std::cout << "Done testing length." << std::endl;
}
<file_sep>/String/test_equal.cpp
// <NAME>
// TESTS: Equality operator
#include "string.hpp"
#include <iostream>
#include <cassert>
int main(){
{
// SETUP
const char s1[1] = { '\0' };
String s2;
//VERIFY
assert(s1 == s2);
assert(s2 == s1);
}
{
// SETUP
const char s1[6] = { '1', '2', '3', '4', 'a'};
String s2(s1);
//VERIFY
std::cout << "1234a == " << s2 << std::endl;
assert(s1 == s2);
assert(s2 == s1);
}
{
// SETUP
const char s1[2] = { 'e' };
String s2(s1);
//VERIFY
std::cout << "e == " << s2 << std::endl;
assert(s1 == s2);
assert(s2 == s1);
}
{
// SETUP
const char s1[9] = { 'e', '3', '1', 'q', 'x', 'y', '/', ' ' };
String s2(s1);
//VERIFY
std::cout << "e31qxy/ == " << s2 << std::endl;
assert(s1 == s2);
assert(s2 == s1);
}
{
// SETUP
char ch = '\0';
String s;
// VERIFY
assert(ch == s);
assert(s == ch);
}
{
// SETUP
char ch = ' ';
String s(ch);
// VERIFY
assert(ch == s);
assert(s == ch);
}
{
// SETUP
char ch = '%';
String s(ch);
// VERIFY
assert(ch == s);
assert(s == ch);
std::cout << "% == " << s << std::endl;
}
{
// SETUP
char ch = 'z';
String s(ch);
// VERIFY
assert(ch == s);
assert(s == ch);
std::cout << "z == " << s << std::endl;
}
{
// SETUP
char ch = '0';
String s(ch);
// VERIFY
assert(ch == s);
assert(s == ch);
std::cout << "0 == " << s << std::endl;
}
std::cout << "Done testing equality." << std::endl;
}
<file_sep>/README.md
# <NAME>
# Kent State University
# Projects Completed for Course
# CS II Data Structures | Spring 2016
<file_sep>/Big Integer/bigint.cpp
/**
* <NAME>
* bigint.cpp
* CS23001
*/
// Defines member funtions of the bigint class
#include "bigint.hpp"
#include <iostream>
// Default Constructor
// Initializes the bigint to 0
bigint::bigint(){
for(int i = 0; i < MAX_SIZE; ++i)
bigintArray[i] = 0;
}
// Int Constructor
// Takes an int and puts it into a bigint
bigint::bigint(int num){
int temp = num;
for(int i = 0; i < MAX_SIZE; ++i){
bigintArray[i] = temp % 10; // Gets the last digit in the int and adds it
temp /= 10; // to the bigint then removes the last digit from the int
}
}
// Character String Constructor
// Takes a string of characters and puts them into a bigint
bigint::bigint(const char charArray[]){
int size = 0;
while(charArray[size] != '\0') // Gets the size of the character array
++size;
for(int i = 0; i < size; ++i)
bigintArray[i] = int(charArray[size - 1 - i]) - int('0'); // Puts the charater array into the bigint
for(int i = size; i < MAX_SIZE; ++i)
bigintArray[i] = 0; // Fills the rest of the bigint with zeros
}
// Addition Operator
// Adds two bigint's together and returns the resulting bingint
bigint bigint::operator+(const bigint& rhs)const{
bigint result;
int digitSum = 0;
bool carryOne = false;
for(int i = 0; i < MAX_SIZE; ++i){
if(carryOne) // If carryOne is true the previous digitSum was >= 10 thus we must carry a one to this sum
digitSum = bigintArray[i] + rhs.bigintArray[i] + 1;
else
digitSum = bigintArray[i] + rhs.bigintArray[i];
result.bigintArray[i] = digitSum % 10;
if(digitSum >= 10)// If digitSum >= 10 then we must carry a 1 to the next digitSum
carryOne = true;
else
carryOne = false;
}
return result;
}
// Multiplication Operator
// Multiplies two bigint's together and returns the resulting bigint
bigint bigint::operator*(const bigint& rhs)const{
bigint product;
bigint temp;
for(int i = 0; i < MAX_SIZE; ++i){
temp = this->timesDigit(rhs.bigintArray[i]);
product = product + temp.times10(i);
}
return product;
}
// Times Digit
// Multiplies a bigint by a single digit int
bigint bigint::timesDigit(int num)const{
bigint result;
if(num == 0)
return result;
if(num == 1)
return *this;
int carry = 0;
int product = 0;
for(int i = 0; i < MAX_SIZE; ++i){
if(carry != 0)// When carry is greater than zero we must add to the next multiplication
product = bigintArray[i] * num + carry;
else
product = bigintArray[i] * num;
result.bigintArray[i] = product % 10;// Gets the last digit of product
carry = product / 10;// Gets the first digit of product
}
return result;
}
// Times Ten
// Multiplies a bigint by 10^i
bigint bigint::times10(int num)const{
bigint product;
if(num == 0)// 10^0 = 1
return *this;
for(int i = 0; i < MAX_SIZE; ++i){
if(i < num)// When i < num we must insert zeros
product.bigintArray[i] = 0;
else// Otherwise we must put the bigint back into the array
product.bigintArray[i] = this->bigintArray[i - num];
}
return product;
}
// Equality Operator
// Checks if two bigints are equal and returns true if so otherwise returns false
bool bigint::operator==(const bigint& rhs)const{
for(int i = 0; i < MAX_SIZE; ++i){
if(bigintArray[i] != rhs.bigintArray[i])
return false;
}
return true;
}
// Subscript Operator
// Returns the digit at index i in bigintArray
int bigint::operator[](int i)const{ return bigintArray[i]; }
// Output operator
// Writes bigints to an output stream
std::ostream& operator<<(std::ostream& os, const bigint& bi){
int digits = 0;
bool atNum = false;
for(int i = MAX_SIZE-1; i > -1; --i){
if(bi.bigintArray[i] != 0) // If we have reached a nonzero digit we are at the number
atNum = true; // that needs to be put into the output stream
if(atNum){
os << bi.bigintArray[i];
++digits; // When we begin adding to the output stream we must
} // keep track of the number of digits in the stream.
if(digits == 50){ // When the number of digits is 50 we must start a new line.
os << std::endl;
digits = 0;
}
}
if(!atNum)// If no nonzero digits where found then bigint is zero
os << 0;
return os;
}
// Input Operator
// Takes data from an input stream and puts it into a bigint
std::istream& operator>>(std::istream& is, bigint& bi){
char temp[MAX_SIZE];
for(int i = 0; i < MAX_SIZE; ++i){
char ch;
is >> ch;
if(ch != ';')
temp[i] = ch;
else{ //If we are at the end of the number we must break out of the loop
temp[i] = '\0';
break;
}
}
bi = bigint(temp);
return is;
}
<file_sep>/Assembler/stack.hpp
// <NAME>
// CS23001
// stack.hpp
// Description: Template defenition for a dynamic stack
// CLASS INV: TOS -> A -> B -> ... -> 0
#include <new>
#ifndef STACK_HPP
#define STACK_HPP
#include "node.hpp"
#include <cassert>
template<typename T>
class Stack{
public:
Stack(): tos(0){};
~Stack();
Stack(Stack<T>&&);
Stack(const Stack<T>&);
Stack<T>& operator=(const Stack<T>&);
Stack<T>& operator=(Stack<T>&&);
void swap(Stack<T>&);
bool isEmpty() const{ return tos == 0; }
bool isFull() const;
T pop();
void push(const T&);
private:
node<T> *tos;
};
// FUNCTION DEFENITIONS
// POP
// REQUIRES: Stack cannot be empty
// ENSURES: tos is removed, and new tos is set
template<typename T>
T Stack<T>::pop(){
assert(tos != 0); // Check stack isn't empty
T result = tos->data;
node<T> *temp = tos; // Keep track of old tos to de-allocate(avoid mem-leak)
tos = tos->next;
delete temp;
return result;
}
// PUSH
// REQUIRES: tos -> A -> B -> ... -> 0
// ENSURES: tos -> x -> A -> B -> ... -> 0
template<typename T>
void Stack<T>::push(const T& x){
assert(!isFull());
node<T> *temp = new node<T>(x);
temp->next = tos; // Make temp's next point to tos
tos = temp; // temp is the new tos
}
// DESTRUCTOR
// REQUIRES:
// ENSURES: All memony in the stack is de-allocated
template<typename T>
Stack<T>:: ~Stack(){
node<T> *temp;
while(tos != 0){ // While we are not at the bottom of the stack
temp = tos;
tos = tos->next;
delete temp;
}
}
// COPY CONSTRUCTOR
// REQUIRES:
// ENSURES: temp is an exact copy of actual
template<typename T>
Stack<T>::Stack(const Stack<T>& actual){
node<T> *temp = actual.tos, *bottom = 0;
tos = 0;
while(temp != 0){
if(tos == 0){ // If we are at the tos
tos = new node<T>(temp->data);
bottom = tos;
}
else{ // If we have more than one node
bottom->next = new node<T>(temp->data);
bottom = bottom->next;
}
temp = temp->next; // Increment temp
}
}
// ASSIGNMENT OPERATOR
// REQUIRES:
// ENSURES: rhs is copied to this
template<typename T>
Stack<T>& Stack<T>::operator=(const Stack<T>& rhs){
if(tos == rhs.tos) // If stacks are the same return
return *this;
node<T> *temp;
while(tos != 0){ // Deallocate everything in this
temp = tos;
tos = tos->next;
delete temp;
}
temp = rhs.tos;
node<T> *bottom = 0;
while(temp != 0){ // Copy rhs to this
if(tos == 0){
tos = new node<T>(temp->data);
bottom = tos;
}
else{
bottom->next = new node<T>(temp->data);
bottom = bottom->next;
}
temp = temp->next;
}
return *this;
}
// SWAP
// REQUIRES:
// ENSURES: this = rhs, rhs = temp(this)
template<typename T>
void Stack<T>::swap(Stack<T> & rhs){
node<T> *temp = tos;
tos = rhs.tos;
rhs.tos = temp;
}
// MOVE ASSIGNMENT
// REQUIRES:
// ENSURES: this = rhs, rhs = this
template<typename T>
Stack<T>& Stack<T>::operator=(Stack<T>&& rhs){
swap(rhs);
return *this;
}
// MOVE CONSTRUCTOR
// REQUIRES:
// ENSURES:
template<typename T>
Stack<T>::Stack(Stack<T>&& actual){
tos = actual.tos;
actual.tos = 0;
}
// ISFULL
// REQUIRES:
// ENSURES: returns true if stack is full, false otherwise
template<typename T>
bool Stack<T>::isFull()const{
node<T> *temp = new(std::nothrow)node<T>();
if(temp != 0){ // If there was enough memory for one more node object
delete temp;
return false;
}
else // No memory left
return true;
}
#endif
<file_sep>/String/test_add.cpp
// <NAME>
// TESTS: Addition
#include "string.hpp"
#include <iostream>
#include <cassert>
int main(){
{
// SETUP
String lhs;
String rhs;
String result;
// TEST
result = lhs + rhs;
// VERIFY
assert(result == '\0');
}
{
// SETUP
String lhs("a");
String rhs("a");
String result;
// TEST
result = lhs + rhs;
// VERIFY
assert(result == "aa");
std::cout << lhs << " + " << rhs << " = " << result << std::endl;
}
{
// SETUP
String lhs;
String rhs('a');
String result;
// TEST
result = lhs + rhs;
// VERIFY
assert(result == 'a');
std::cout << lhs << " + " << rhs << " = " << result << std::endl;
}
{
// SETUP
String lhs("abc");
String rhs("def");
String result;
// TEST
result = lhs + rhs;
// VERIFY
assert(result == "abcdef");
std::cout << lhs << " + " << rhs << " = " << result << std::endl;
}
{
// SETUP
String lhs(" ");
String rhs(" ");
String result;
// TEST
result = lhs + rhs;
// VERIFY
assert(result == " ");
}
{
// SETUP
String lhs("DANK");
String rhs(" MEMES");
String result;
// TEST
result = lhs + rhs;
// VERIFY
assert(result == "DANK MEMES");
std::cout << lhs << " + " << rhs << " = " << result << std::endl;
}
{
// SETUP
String lhs("Flowy, Toriel, Sans, Payparus, Undyne, Alphys, ");
String rhs("Mettaton, Asgore, <NAME>.");
String result;
// TEST
result = lhs + rhs;
// VERIFY
assert(result == "Flowy, Toriel, Sans, Payparus, Undyne, Alphys, Mettaton, Asgore, Asriel, Chara.");
std::cout << lhs << " + " << rhs << " = " << result << std::endl;
}
{
// SETUP
String lhs("OOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOO");
String rhs("OOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOO");
String result;
// TEST
result = lhs + rhs;
// VERIFY
assert(result == "OOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOO");
}
{
// SETUP
String lhs("Wow that passes.");
String rhs(" ~!@#$%^&*())?GHDFBN:_SE+GH'dghokwet][]");
String result;
// TEST
result = lhs + rhs;
// VERIFY
assert(result == "Wow that passes. ~!@#$%^&*())?GHDFBN:_SE+GH'dghokwet][]");
std::cout << lhs << " + " << rhs << " = " << result << std::endl;
}
{
// SETUP
String lhs("And so I cry somtimes when I'm lying in bed Just to get it all out what's in my head and I, I am feeling a little peculiar And so I wake in the morning and I step outside And I take A deep breath and I get real high And I scream from the top of my lungs, What's goin on");
String rhs(" And I say HEYAYAYAYAYAY HEYAYA I said hey, what's goin' on And I say HEYAYAYAYA HEYAYA I said hey, what's goin' on");
String result;
// TEST
result = lhs + rhs;
// VERIFY
assert(result == "And so I cry somtimes when I'm lying in bed Just to get it all out what's in my head and I, I am feeling a little peculiar And so I wake in the morning and I step outside And I take A deep breath and I get real high And I scream from the top of my lungs, What's goin on And I say HEYAYAYAYAYAY HEYAYA I said hey, what's goin' on And I say HEYAYAYAYA HEYAYA I said hey, what's goin' on");
}
{
// SETUP
char ch = '1';
const String s;
String result;
// TEST
result = ch + s;
// VERIFY
assert(result == '1');
result = s + ch;
assert(result == '1');
}
{
// SETUP
const char ch = '1';
const String s('a');
String result;
// TEST
result = ch + s;
// VERIFY
assert(result == "1a");
result = s + ch;
assert(result == "a1");
}
{
// SETUP
char ch = '0';
const String s(" TESTS");
String result;
// TEST
result = ch + s;
// VERIFY
assert(result == "0 TESTS");
result = s + ch;
assert(result == " TESTS0");
}
{
// SETUP
const char c[DEFAULT_STRING_CAPACITY] = { 'a' };
String s;
String result;
// TEST
result = c + s;
// VERIFY
assert(result == 'a');
result = s + c;
assert(result == 'a');
}
{
// SETUP
const char c[DEFAULT_STRING_CAPACITY] = { 'a' };
String s("1");
String result;
// TEST
result = c + s;
// VERIFY
assert(result == "a1");
result = s + c;
assert(result == "1a");
}
{
// SETUP
const char c[DEFAULT_STRING_CAPACITY] = { 'a', 'b', 'c' };
String s("def");
String result;
// TEST
result = c + s;
// VERIFY
assert(result == "abcdef");
result = s + c;
assert(result == "defabc");
}
std::cout << "Done testing addition." << std::endl;
}
<file_sep>/Big Integer/test_add.cpp
// bigint Test Program
//
// Tests: int constructor, uses ==
//
#include <iostream>
#include <cassert>
#include "bigint.hpp"
//===========================================================================
int main () {
{
//------------------------------------------------------
// Setup fixture
bigint left(0);
bigint right(0);
bigint result;
// Test
result = left + right;
// Verify
assert(left == 0);
assert(right == 0);
assert(result == 0);
}
{
//------------------------------------------------------
// Setup fixture
bigint left(1);
bigint right(0);
bigint result;
// Test
result = left + right;
// Verify
assert(left == 1);
assert(right == 0);
assert(result == 1);
}
{
//------------------------------------------------------
// Setup fixture
bigint left(1);
bigint right(1);
bigint result;
// Test
result = left + right;
// Verify
assert(left == 1);
assert(right == 1);
assert(result == 2);
}
{
//------------------------------------------------------
// Setup fixture
bigint left(9);
bigint right(9);
bigint result;
// Test
result = left + right;
// Verify
assert(left == 9);
assert(right == 9);
assert(result == 18);
}
{
//------------------------------------------------------
// Setup
bigint left(19);
bigint right(19);
bigint result;
// Test
result = left + right;
// Verify
assert(left == 19);
assert(right == 19);
assert(result == 38);
}
{
//------------------------------------------------------
// Setup
bigint left(199);
bigint right(199);
bigint result;
// Test
result = left + right;
// Verify
assert(left == 199);
assert(right == 199);
assert(result == 398);
}
{
//------------------------------------------------------
// Setup
bigint left(11111);
bigint right("99999999999999999999999999999999999999999999999999");
bigint result;
// Test
result = left + right;
// Verify
assert(left == 11111);
assert(right == "99999999999999999999999999999999999999999999999999");
assert(result == "100000000000000000000000000000000000000000000011110");
}
{
//------------------------------------------------------
// Setup
bigint left("1879465321012279853475324858234852345723845823458");
bigint right("123514959195931495934959234959293459923459923495");
bigint result;
// Test
result = left + right;
// Verify
assert(left == "1879465321012279853475324858234852345723845823458");
assert(right == "123514959195931495934959234959293459923459923495");
assert(result == "2002980280208211349410284093194145805647305746953");
}
{
//------------------------------------------------------
// Setup
bigint left("6666666666666666666666666666666666666666666666666666666666666666666666666666666666666666666666666666");
bigint right("55555555555555555555555555555555555555555555555555");
bigint result;
// Test
result = left + right;
// Verify
assert(left == "6666666666666666666666666666666666666666666666666666666666666666666666666666666666666666666666666666");
assert(right == "55555555555555555555555555555555555555555555555555");
assert(result == "6666666666666666666666666666666666666666666666666722222222222222222222222222222222222222222222222221");
}
//Add test cases as needed.
std::cout << "Done with testing addition." << std::endl;
}
<file_sep>/Assembler/test_default_ctor.cpp
// <NAME>
// TESTS: Default Constructor & IsEmpty
#include "string/string.hpp"
#include "stack.hpp"
#include <iostream>
int main(){
{
//TEST
Stack<int> s;
//VERIFY
assert(s.isEmpty());
}
{
//TEST
Stack<String> s;
//VERIFY
assert(s.isEmpty());
}
{
//TEST
Stack<char> s;
//VERIFY
assert(s.isEmpty());
}
{
//TEST
Stack<double> s;
//VERIFY
assert(s.isEmpty());
}
{
//TEST
Stack<bool> s;
//VERIFY
assert(s.isEmpty());
}
{
//TEST
Stack<long> s;
//VERIFY
assert(s.isEmpty());
}
{
//TEST
Stack<float> s;
//VERIFY
assert(s.isEmpty());
}
{
//TEST
Stack<short> s;
//VERIFY
assert(s.isEmpty());
}
std::cout << "Done testing default constructor." << std::endl;
}
<file_sep>/String/test_relationaloperators.cpp
// <NAME>
// TESTS: <, <=, >, >=, !=
#include "string.hpp"
#include <iostream>
#include <cassert>
int main(){
{
// TEST
String s1('a');
String s2('c');
// VERIFY
assert(s1 < s2);
}
{
// TEST
String s1("accept");
String s2("access");
String s3("acceptance");
// VERIFY
assert(s1 < s2);
assert(s3 < s2);
assert(s1 < s3);
}
{
// TEST
String s1("aa");
String s2("aaa");
// VERIFY
assert(s1 < s2);
}
{
// TEST
String s1("9");
String s2("10");
// VERIFY
assert(s2 < s1);
}
{
// TEST
String s1("a");
String s2("A");
// VERIFY
assert(s2 < s1);
}
{
// TEST
const char s1('a');
String s2('c');
// VERIFY
assert(s1 < s2);
}
{
// TEST
const char s1[7] = {'a', 'c', 'c', 'e', 's', 's'};
String s2("accept");
// VERIFY
assert(s2 < s1);
}
{
// TEST
const char s1[3] = { 'a', 'a'};
String s2("aaa");
// VERIFY
assert(s1 < s2);
}
{
// TEST
const char ch = 'a';
String s('c');
// VERIFY
assert(ch < s);
}
{
// TEST
const char ch = '1';
String s('9');
// VERIFY
assert(ch < s);
}
{
// TEST
String lhs("a");
String rhs("a");
// VERIFY
assert(lhs <= rhs);
}
{
// TEST
String lhs("abc");
String rhs("abc");
// VERIFY
assert(lhs <= rhs);
}
{
// TEST
String lhs("a");
String rhs("c");
// VERIFY
assert(lhs <= rhs);
}
{
// TEST
String lhs("a");
String rhs("c");
// VERIFY
assert(lhs != rhs);
}
{
// TEST
String lhs("a");
String rhs(" a");
// VERIFY
assert(lhs != rhs);
}
{
// TEST
String lhs;
String rhs("c");
// VERIFY
assert(lhs != rhs);
}
{
// TEST
String lhs("a b c");
String rhs("abc");
// VERIFY
assert(lhs != rhs);
}
{
// TEST
String lhs("access");
String rhs("accept");
// VERIFY
assert(lhs != rhs);
}
{
// TEST
String lhs("c");
String rhs("a");
// VERIFY
assert(lhs >= rhs);
}
{
// TEST
String lhs("a");
String rhs("a");
// VERIFY
assert(lhs >= rhs);
}
{
// TEST
String lhs("abc");
String rhs("abc");
// VERIFY
assert(lhs >= rhs);
}
{
// TEST
String lhs("access");
String rhs("accept");
// VERIFY
assert(lhs >= rhs);
}
{
// TEST
String lhs("c");
String rhs("a");
// VERIFY
assert(lhs > rhs);
}
{
// TEST
String lhs("c");
String rhs("a");
// VERIFY
assert(lhs > rhs);
}
{
// TEST
String lhs("access");
String rhs("accept");
// VERIFY
assert(lhs > rhs);
}
{
// TEST
String lhs("9");
String rhs("0");
// VERIFY
assert(lhs > rhs);
}
std::cout << "Done testing relational operators." << std::endl;
}
<file_sep>/String/test_default_ctor.cpp
// String class test program
// TESTS: Default Constuctor
#include <iostream>
#include <cassert>
#include "string.hpp"
int main(){
{
//SETUP
//TEST
String str;
//VERIFY
assert(str == '\0');
}
std::cout << "Done testing default ctor." << std::endl;
}
<file_sep>/Assembler/node.hpp
// <NAME>
// CS23001
// node.hpp
// Description: Defenition for node object
#ifndef NODE_HPP
#define NODE_HPP
template <typename T>
class node{
public:
T data;
node<T> *next;
node(): next(0){};
node(const T& x): data(x), next(0){};
};
#endif
<file_sep>/String/test_cap_ctor.cpp
// <NAME>
// TESTS: Capacity Constructors
#include "string.hpp"
#include <iostream>
#include <cassert>
int main(){
{
// SETUP
String s(1);
// VERIFY
assert( 1 == s.capacity());
}
{
// SETUP
String s(1);
// VERIFY
assert( 1 == s.capacity());
assert(s == '\0');
}
{
// SETUP
String s(56);
// VERIFY
assert( 56 == s.capacity());
assert(s == '\0');
}
{
// SETUP
String s(300);
// VERIFY
assert( 300 == s.capacity());
assert(s == '\0');
}
{
// SETUP
String s(1000);
// VERIFY
assert( 1000 == s.capacity());
assert(s == '\0');
}
std::cout << "Done testing capacity constructors." << std::endl;
}
<file_sep>/String/test_split.cpp
// <NAME>
// TESTS: Split
#include <iostream>
#include <cassert>
#include "string.hpp"
int main(){
{
//SETUP
String s;
std::vector<String> result;
//TEST
result = s.split(' ');
//VERIFY
assert(result[0] == s);
assert(result.size() == 1);
}
{
//SETUP
String s("computer");
std::vector<String> result;
//TEST
result = s.split('p');
//VERIFY
assert(result[0] == "com");
assert(result[1] == "uter");
assert(result.size() == 2);
}
{
//SETUP
String s("abc ef gh");
std::vector<String> result;
//TEST
result = s.split(' ');
//VERIFY
assert(result[0] == "abc");
assert(result[1] == "ef");
assert(result[2] == "gh");
assert(result.size() == 3);
}
{
//SETUP
String s("abc ef gh");
std::vector<String> result;
//TEST
result = s.split(' ');
//VERIFY
assert(result[0] == "abc");
assert(result[1] == "ef");
assert(result[2] == "gh");
assert(result.size() == 3);
}
{
//SETUP
String s("ab c de");
std::vector<String> result;
//TEST
result = s.split(' ');
//VERIFY
assert(result[0] == "ab");
assert(result[1] == "c");
assert(result[2] == "de");
assert(result.size() == 3);
}
{
//SETUP
String s("ab c de");
std::vector<String> result;
//TEST
result = s.split(' ');
//VERIFY
assert(result[0] == "ab");
assert(result[1] == "c");
assert(result[2] == "de");
assert(result.size() == 3);
}
{
//SETUP
String s("c");
std::vector<String> result;
//TEST
result = s.split(' ');
//VERIFY
assert(result[0] == "c");
assert(result.size() == 1);
}
{
//SETUP
String s(" ab c");
std::vector<String> result;
//TEST
result = s.split(' ');
//VERIFY
assert(result[0] == "ab");
assert(result[1] == "c");
assert(result.size() == 2);
}
{
//SETUP
String s("abc ");
std::vector<String> result;
//TEST
result = s.split(' ');
//VERIFY
assert(result[0] == "abc");
assert(result.size() == 1);
}
{
//SETUP
String s("a b c ");
std::vector<String> result;
//TEST
result = s.split(' ');
//VERIFY
assert(result[0] == "a");
assert(result[1] == "b");
assert(result[2] == "c");
assert(result.size() == 3);
}
{
//SETUP
String s(" ");
std::vector<String> result;
//TEST
result = s.split(' ');
//VERIFY
assert(result.size() == 0);
}
{
//SETUP
String s("abc");
std::vector<String> result;
//TEST
result = s.split(' ');
//VERIFY
assert(result[0] == "abc");
assert(result.size() == 1);
}
{
//SETUP
String s("S Sp Spa Spac Space Spac");
std::vector<String> result;
//TEST
result = s.split(' ');
//VERIFY
assert(result[0] == "S");
assert(result[1] == "Sp");
assert(result[2] == "Spa");
assert(result[3] == "Spac");
assert(result[4] == "Space");
assert(result[5] == "Spac");
assert(result.size() == 6);
}
std::cout << "Done testing split." << std::endl;
}
<file_sep>/String/test_subscript.cpp
// <NAME>
// TESTS: Subscript operator
#include "string.hpp"
#include <iostream>
#include <cassert>
int main(){
{
// TEST
String s;
// VERIFY
assert(s[0] == '\0');
}
{
// TEST
String s('a');
// VERIFY
assert(s[0] == 'a');
}
{
// TEST
String s("abc123");
// VERIFY
assert(s[5] == '3');
}
{
// TEST
String s(" ");
// VERIFY
assert(s[3] == ' ');
}
{
// TEST
String s("1000000000000000000000000000000000000000000000000000000000001");
// VERIFY
assert(s[60] == '1');
}
{
// TEST
String s("Tests tests tests test test test. test %#$%#$%#$%#%#$%");
// VERIFY
assert(s[27] == 't');
}
{
// TEST
String s("WOOOOOOOOOOOOOOOOOOoooOOOoOOOoooOOoooOOOoOOOooooOOOOOOOoOOOOOOooooOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOoooOooOOOOOOOOOOooOOOOOOOOOOOOOOOOOOOOOOOOoO");
// VERIFY
assert(s[100] == 'o');
}
std::cout << "Done testing subscript." << std::endl;
}
<file_sep>/String/string.cpp
// <NAME>
// CS 23001
// string.cpp
// Defines funtions from string.hpp
#include <iostream>
#include "string.hpp"
#include <cassert>
// Default Constructor
// Initializes the pointer s and Capacity to 256
String::String(){
Capacity = DEFAULT_STRING_CAPACITY;
s = new char[Capacity];
s[0] = '\0';
}
// Character Constructor
// Initializes the pointer s to a character and Capacity to 256
String::String(char ch){
Capacity = DEFAULT_STRING_CAPACITY;
s = new char[Capacity];
s[0] = ch;
s[1] = '\0';
}
// Character Array Constructor
// Initializes the pointer s to a character array and Capacity to 256
String::String(const char *str){
Capacity = DEFAULT_STRING_CAPACITY;
s = new char[Capacity];
int i = 0;
while(str[i] != '\0'){
s[i] = str[i];
++i;
if(i >= capacity()-1)
resetCapacity(capacity()*2);
}
s[i] = '\0';
}
// Capacity and Character Array Constructor
// Initializes s to a character array and Capacity to a given Capacity n
String::String(int n, const char *str){
assert(n > 0); // A pointer to an array of size 0 is undefined
Capacity = n;
s = new char[Capacity];
int i = 0;
while(str[i] != '\0'){
s[i] = str[i];
++i;
}
s[i] = '\0';
}
// Capacity Constructor
// Initializes string to a given Capacity n
String::String(int n){
assert(n > 0); // A pointer to an array of size 0 is undefined
Capacity = n;
s = new char[Capacity];
s[0] = '\0';
}
// Destructor
// Deallocates memory in String
String::~String(){ delete[] s; }
// Copy Constructor
// Makes temporary copies of this
String::String(const String& actual){
Capacity = actual.Capacity;
s = new char[Capacity];
for(int i = 0; i < Capacity; ++i)
s[i] = actual.s[i];
}
// Assignment operator
// Sets this equal to rhs
String& String::operator=(String rhs){
swap(rhs);
return *this;
}
// Swap
// Constant time swap
void String::swap(String& rhs){
char *temp = s;
s = rhs.s;
rhs.s = temp;
int ctemp = Capacity;
Capacity = rhs.Capacity;
rhs.Capacity = ctemp;
}
// resetCapacity
// Changes the capacity of s to n
void String::resetCapacity(int n){
assert(n > -1);
char *temp = new char[n];
Capacity = n;
if(n == 0){// If n is zero return an empty character array
delete[] s;
s = temp;
}
else{
int i = 0;
while(s[i] != '\0' && i < n - 1){
temp[i] = s[i];
++i;
}
temp[i] = '\0';
delete[] s;
s = temp;
}
}
// Output Operator
// Displays the String
std::ostream& operator<<(std::ostream& out, const String &rhs){
out << rhs.s;
return out;
}
// Input Operator
// Reads input one word at a time
std::istream& operator>>(std::istream &in, String &rhs){
char ch = '@';
int newCap = 0;
rhs = "";
while(ch != ' ' && ch != '\n' && ch != '\t' && ch != '\v' && ch != '\r' && ch != '\f' && !in.eof()){// Run while ch != whitespace and end of file
in.get(ch);
if((ch == ' ' || ch == '\n' || ch== '\t') && rhs == ""){// Ensures that rhs is never null, moves past extra spaces
while(ch == ' ' || ch == '\n' || ch == '\t')
in.get(ch);
}
if(rhs.length() >= rhs.capacity()){// Resize input when it reached capacity
newCap = rhs.capacity() * 2;
rhs.resetCapacity(newCap);
}
if(ch != ' ' && ch != '\n' && ch != '\t' && ch != '\r' && ch != '\f' && ch != '\v')// Add ch to temp if it is not whitespace
rhs = rhs + ch;
}
rhs[rhs.capacity()-1] = '\0';
return in;
}
// Addtition Operators
// Combines two Strings
String String::operator+(const String &rhs)const{
int newCap = DEFAULT_STRING_CAPACITY;
if(DEFAULT_STRING_CAPACITY <= length() + rhs.length()){// If the combined lengths are greater than the default cap, result needs a new capacity
while(newCap <= length() + rhs.length())
newCap = newCap + DEFAULT_STRING_CAPACITY;
}
String result(newCap, s);
int offset = length();
int i = 0;
while(rhs.s[i] != '\0'){
result.s[offset + i] = rhs.s[i]; // Starts at the end of this and adds rhs
++i;
}
result.s[offset + i] = '\0';
return result;
}
String operator+(const char lhs[], const String &rhs){ return String(lhs) + rhs; }
String operator+(const char lhs, const String &rhs){ return String(lhs) + rhs; }
// Substring
// Returns a substring between a given starting and ending index
String String::substr(int start, int end)const{
String result;
assert(0 <= start);
assert(start <= end);
assert(end < length());
for(int i = start; i <= end; ++i)
result.s[i - start] = s[i]; // Gets the substring out of string
result.s[end - start + 1] = '\0';
return result;
}
// Find String
// Looks for a substring inside a String and return true if found
bool String::findstr(const String &find)const{
if(find.length() == 1 && s[0] == find.s[0])// If find is one char and == this then just return true
return true;
char start = find.s[0];
char end = find.s[find.length()-1];
bool search = false;
bool found = false;
int startI = 0;
for(int i = 0; i < length(); ++i){
if(s[i] == start) // If find the first char we must start looking at the next chars
search = true;
if(search && s[i] != find.s[startI]){// If we are looking at the substring and the chars do not match then our search was not succesful
search = false;
startI = 0;
}
if(search && s[i] == find.s[startI]) // Increment startI if we find another char consistent with find
++startI;
if(search && s[i] == end && startI == find.length())// If we reach the last char in find then we have found the substring in this
return true;
}
return found;
}
// Find Char
// Looks for a character in a String and returns true if found
bool String::findchar(const char &find)const{
for(int i = 0; i < length(); ++i){
if(s[i] == find)
return true;
}
return false;
}
// Equality Operators
// Checks if two String's are equal
bool String::operator==(const String &rhs)const{
int i = 0;
while((s[i] != '\0') && (s[i] == rhs.s[i]))
++i;
return s[i] == rhs.s[i];
}
bool operator==(const char s[], const String &lhs){ return lhs == s; }
bool operator==(const char ch, const String &lhs){ return lhs == ch; }
// Subscript Operators
// Returns the value at index i in the array
char String::operator[](int i)const{
assert(i >= 0);
assert(i < length());
return s[i];
}
char& String::operator[](int i){return s[i]; }
// Other Relational Operators
// Compares Strings
bool String::operator<(const String &rhs)const{
int i = 0;
while((s[i] != '\0') && (rhs.s[i] != '\0') && (s[i] == rhs.s[i]))
++i;
return s[i] < rhs.s[i];
}
bool operator<(const char s[], const String &s1){ return !(s1 < s); }
bool operator<(const char ch, const String &s1){ return !(s1 < ch); }
bool operator<=(const String &lhs, const String &rhs){ return ((lhs == rhs) || (lhs < rhs)); }
bool operator!=(const String &lhs, const String &rhs){ return !(lhs == rhs); }
bool operator>=(const String &lhs, const String &rhs){ return ((lhs == rhs) || !(lhs < rhs)); }
bool operator>(const String &lhs, const String &rhs){ return !(lhs < rhs); }
// Length
// Returns the length of the String
int String::length()const{
int L = 0;
while(s[L] != '\0')
++L;
return L;
}
// Capacity
// Returns the Capacity of the String
int String::capacity()const{ return Capacity; }
// Split
// Returns a vector of Strings split at a given char
std::vector<String> String::split(char ch) const{
std::vector<String> vecS;
if(!(findchar(ch))){ // If ch is not in the string, return the string
vecS.push_back(*this);
return vecS;
}
String temp;
for(int i = 0; i < length(); ++i){
if(s[i] != ch){ //If we are not at ch add the character to the temporary string
temp = temp + s[i];
}
if(s[i] == ch && temp != '\0'){ // If we are at ch and the temporary string is not null
vecS.push_back(temp); // Add the temp string to the vector
temp = ""; // Reset the temp string to an empty string
}
}
if(temp != '\0')//Add temp to the vector when we reach the end of the string
vecS.push_back(temp);
return vecS;
}
<file_sep>/Assembler/test_assignment.cpp
// <NAME>
// TESTS: Assignmnet operator
#include "stack.hpp"
#include "string/string.hpp"
#include <iostream>
int main(){
{
//SETUP
Stack<int> s1;
Stack<int> s2;
//TEST
s1 = s2;
assert(s1.isEmpty());
assert(s2.isEmpty());
s2 = s1;
assert(s1.isEmpty());
assert(s2.isEmpty());
}
{
//SETUP
Stack<int> s1;
Stack<int> s2;
s1.push(1);
//TEST
s2 = s1;
assert(s2.pop() == 1);
assert(s2.isEmpty());
s1 = s2;
assert(s1.isEmpty());
assert(s2.isEmpty());
}
{
//SETUP
Stack<int> s1;
Stack<int> s2;
s1.push(1);
s1.push(10);
s1.push(100);
s2.push(1);
//TEST
s2 = s2;
assert(s2.pop() == 1);
assert(s2.isEmpty());
s2 = s1;
assert(s2.pop() == 100);
assert(s2.pop() == 10);
assert(s2.pop() == 1);
assert(s2.isEmpty());
s1 = s2;
assert(s1.isEmpty());
assert(s2.isEmpty());
}
{
//SETUP
Stack<String> s1;
Stack<String> s2;
//TEST
s1 = s2;
assert(s1.isEmpty());
assert(s2.isEmpty());
s2 = s1;
assert(s1.isEmpty());
assert(s2.isEmpty());
}
{
//SETUP
Stack<String> s1;
Stack<String> s2;
s1.push("");
s2.push("");
//TEST
s1 = s2;
assert(s2.pop() == "");
assert(s2.isEmpty());
s1 = s2;
assert(s1.isEmpty());
assert(s2.isEmpty());
}
{
//SETUP
Stack<String> s1;
Stack<String> s2;
s1.push("a");
s1.push("ab");
s1.push("abc");
s1.push("abcd");
//TEST
s2 = s1;
assert(s2.pop() == "abcd");
assert(s2.pop() == "abc");
assert(s2.pop() == "ab");
assert(s2.pop() == "a");
assert(s2.isEmpty());
s2.push("word");
s2 = s1;
assert(s2.pop() == "abcd");
assert(s2.pop() == "abc");
assert(s2.pop() == "ab");
assert(s2.pop() == "a");
assert(s2.isEmpty());
s1 = s2;
assert(s1.isEmpty());
assert(s2.isEmpty());
}
std::cout << "Done testing assignment." << std::endl;
}
<file_sep>/Big Integer/multiply.cpp
/**
* <NAME>
* multiply.cpp
* CS 23001
*/
// Reads data from files to bigints and multiplies them
#include <iostream>
#include <fstream>
#include <cstdlib>
#include "bigint.hpp"
int main(){
std::ifstream in("data1-2.txt");
if(!in.is_open()){
std::cerr << "Could not open file data1-2.txt, exiting." << std::endl;
exit(1);
}
bigint numOne;
bigint numTwo;
bigint product;
while(!in.eof()){
in >> numOne;
if(in.eof()) break;// Stops output of random data due to whitespace in the end of the file
in >> numTwo;
std::cout << std::endl;
std::cout << "Bigint one is: " << numOne << std::endl << std::endl;
std::cout << "Bigint two is: " << numTwo << std::endl;
product = numOne * numTwo;
std::cout << "The product is: " << product << std::endl;
}
in.close();
return 0;
}
<file_sep>/String/test_ctor_charcap.cpp
// <NAME>
// TESTS: Cap/Character Array Constructor
#include <iostream>
#include "string.hpp"
#include <cassert>
int main(){
{
// SETUP
String s(1, "");
// VERIFY
assert(s == '\0');
assert(1 == s.capacity());
}
{
// SETUP
String s(2, "a");
// VERIFY
assert(s == "a");
assert(2 == s.capacity());
}
{
// SETUP
String s(4, "abc");
// VERIFY
assert(s == "abc");
assert(4 == s.capacity());
}
{
// SETUP
String s(481, "Leedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle Lee");
// VERIFY
assert(s == "Leedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle LeeLeedle Leedle Leedle Lee");
assert(481 == s.capacity());
}
std::cout << "Done testing char cap ctor." << std::endl;
}
| 0eb150aa7f8e38f27c9c4927c0033419f8466c4f | [
"Markdown",
"C++"
] | 37 | C++ | aaliszt/CS-II-Data-Structures | 5ff5dcf4c3cbc59e91a810215792a68208ded31f | 4d4ca4a486fa6a15c890f933dda47a100b337468 | |
refs/heads/master | <file_sep>package ca.ubc.ece.cpen221.mp4.ai;
import java.util.Set;
import ca.ubc.ece.cpen221.mp4.Location;
import ca.ubc.ece.cpen221.mp4.Util;
import ca.ubc.ece.cpen221.mp4.World;
import ca.ubc.ece.cpen221.mp4.chessPieces.ChessPiece;
import ca.ubc.ece.cpen221.mp4.chessPieces.Queen;
import ca.ubc.ece.cpen221.mp4.commands.Command;
import ca.ubc.ece.cpen221.mp4.commands.MoveCommand;
import ca.ubc.ece.cpen221.mp4.commands.WaitCommand;
/**
* An artificial intelligence that allows a ChessPiece with the
* potentialLocations of a Pawn to behave as a Pawn would in a game of chess.
* Once reaching the end of the world Pawn isDead and a Queen spawns in its
* place.
*
*/
public class PawnAI implements ChessAI {
@Override
public Command getNextAction(World world, ChessPiece piece) {
Set<Location> possibleMoves = piece.potentialLocations();
// When the Pawn reaches the top or bottom of the World, remove the pawn
// and spawn a new Queen
if ((piece.getLocation().getY() == 0 || piece.getLocation().getY() == world.getHeight() - 1)
&& !piece.isDead()) {
Location loc = piece.getLocation();
piece.loseEnergy(100);
world.step();
promotionPawn(world, loc);
return new WaitCommand();
}
// Move into one of the Pawn's possible Locations if Empty and Valid
for (Location loc : possibleMoves) {
if (Util.isLocationEmpty(world, loc)) {
return new MoveCommand(piece, loc);
}
}
return new WaitCommand();
}
/**
* Spawns a new Queen in a location that a pawn previously existed
*
* @param world
* the World in which the Queen is to be spawned
* @param loc
* the Location in which to spawn a new Queen, must be Valid and
* Empty
*
*/
private void promotionPawn(World world, Location loc) {
QueenAI queenAI = new QueenAI();
Queen queen = new Queen(queenAI, loc);
world.addItem(queen);
world.addActor(queen);
}
}
<file_sep>package ca.ubc.ece.cpen221.mp4.ai;
import ca.ubc.ece.cpen221.mp4.ArenaWorld;
import ca.ubc.ece.cpen221.mp4.World;
import ca.ubc.ece.cpen221.mp4.chessPieces.ChessPiece;
import ca.ubc.ece.cpen221.mp4.commands.Command;
/**
* The AI interface for all ChessPieces. Each ChessPiece possess a unique
* implementation of this interface
*
*/
public interface ChessAI {
/**
* Returns the next Command that the ChessPiece will perform based on its
* unique behavior
*
* @param world
* the World in which the ChessPiece exists
* @param piece
* a chessPiece, preferably matched to its corresponding AI
* @return the next Command for the piece to perform
*/
public Command getNextAction(World world, ChessPiece piece);
}
<file_sep>package ca.ubc.ece.cpen221.mp4.chessPieces;
import java.util.HashSet;
import java.util.Random;
import java.util.Set;
import javax.swing.ImageIcon;
import ca.ubc.ece.cpen221.mp4.Location;
import ca.ubc.ece.cpen221.mp4.Util;
import ca.ubc.ece.cpen221.mp4.World;
import ca.ubc.ece.cpen221.mp4.ai.ChessAI;
import ca.ubc.ece.cpen221.mp4.commands.Command;
/**
* A Knight implementation of a ChessPieces has potential locations in a
* distance 3 L shape around its current location. It is assigned a random
* colour "black" or "white"
*
*/
public class Knight implements ChessPiece {
// Arbitrary
private static final int INITIAL_ENERGY = 40;
// Arbitrary
private static final int STRENGTH = 200;
// Slower COOLDOWN time
private static final int COOLDOWN = 4;
// Knight moves 3 spaces in its L pattern
private static final int MOVING_RANGE = 3;
private static final ImageIcon knightImage = Util.loadImage("knight.gif");
// Recommended KnightAI
private final ChessAI ai;
private Location location;
private int energy;
// Generate a random number to determine colour
private final int random = new Random().nextInt(10);
public Knight(ChessAI knightAI, Location initialLocation) {
ai = knightAI;
location = initialLocation;
energy = INITIAL_ENERGY;
}
@Override
public void moveTo(Location targetLocation) {
location = targetLocation;
}
@Override
public int getMovingRange() {
return MOVING_RANGE;
}
@Override
public ImageIcon getImage() {
return knightImage;
}
@Override
public String getName() {
return "knight";
}
@Override
public Location getLocation() {
return this.location;
}
@Override
public int getStrength() {
return STRENGTH;
}
@Override
public void loseEnergy(int energyLost) {
energy -= energyLost;
}
@Override
public boolean isDead() {
return energy <= 0;
}
@Override
public int getPlantCalories() {
return 0;
}
@Override
public int getMeatCalories() {
return 0;
}
@Override
public int getCoolDownPeriod() {
return COOLDOWN;
}
@Override
public Command getNextAction(World world) {
Command nextAction = ai.getNextAction(world, this);
return nextAction;
}
/**
* Knight moves in a distance 3 "L" shape in any direction. A set of all the
* locations a Knight could move if they are Valid and Empty
*
* @return a set of all the possible Locations a knight could move if
* Locations are Valid and Empty
*/
@Override
public Set<Location> potentialLocations() {
Set<Location> locations = new HashSet<Location>();
locations.add(new Location(location.getX() + 2, location.getY() + 1));
locations.add(new Location(location.getX() + 2, location.getY() - 1));
locations.add(new Location(location.getX() - 2, location.getY() + 1));
locations.add(new Location(location.getX() - 2, location.getY() - 1));
locations.add(new Location(location.getX() + 1, location.getY() + 2));
locations.add(new Location(location.getX() - 1, location.getY() + 2));
locations.add(new Location(location.getX() + 1, location.getY() - 2));
locations.add(new Location(location.getX() - 1, location.getY() - 2));
return locations;
}
@Override
public String colour() {
if (this.random % 2 == 1) {
return "white";
}
return "black";
}
}
<file_sep>package ca.ubc.ece.cpen221.mp4.items.animals;
import javax.swing.ImageIcon;
import ca.ubc.ece.cpen221.mp4.Food;
import ca.ubc.ece.cpen221.mp4.Location;
import ca.ubc.ece.cpen221.mp4.Util;
import ca.ubc.ece.cpen221.mp4.World;
import ca.ubc.ece.cpen221.mp4.ai.AI;
import ca.ubc.ece.cpen221.mp4.ai.ArenaAnimalAI;
import ca.ubc.ece.cpen221.mp4.ai.PredatorAI;
import ca.ubc.ece.cpen221.mp4.commands.Command;
import ca.ubc.ece.cpen221.mp4.items.LivingItem;
/**
*The {@link Tiger} is an {@link ArenaAnimal} that uses the {@link PredatorAI}.
*/
public class Tiger implements ArenaAnimal {
// Constant attributes of the Tiger implementation of Animal
private static final int INITIAL_ENERGY = 20;
private static final int MAX_ENERGY = 200;
private static final int STRENGTH = 400;
private static final int VIEW_RANGE = 5;
private static final int MIN_BREEDING_ENERGY = 100;
private static final int COOLDOWN = 2;
private static final int MOVING_RANGE = 1;
private static final ImageIcon tigerImage = Util.loadImage("tiger.gif");
private final AI ai;
private Location location;
private int energy;
/**
* Constructs a new Tiger at a certain initialLocation
*
* @param initialLocation
* the location in which the Tiger is created
*/
public Tiger(Location initialLocation) {
// Tiger uses the PredatorAI
this.ai = new PredatorAI();
this.location = initialLocation;
this.energy = INITIAL_ENERGY;
}
@Override
public LivingItem breed() {
Tiger child = new Tiger(location);
child.energy = energy / 2;
this.energy = energy / 2;
return child;
}
@Override
public void eat(Food food) {
energy = Math.min(MAX_ENERGY, energy + food.getMeatCalories());
}
@Override
public int getCoolDownPeriod() {
return COOLDOWN;
}
@Override
public int getEnergy() {
return energy;
}
@Override
public ImageIcon getImage() {
return tigerImage;
}
@Override
public Location getLocation() {
return location;
}
@Override
public int getMaxEnergy() {
return MAX_ENERGY;
}
@Override
public int getMeatCalories() {
// The amount of meat calories it provides is equal to its current
// energy.
return energy;
}
@Override
public int getMinimumBreedingEnergy() {
return MIN_BREEDING_ENERGY;
}
@Override
public int getMovingRange() {
return MOVING_RANGE; // Can only move to adjacent locations.
}
@Override
public String getName() {
return "Tiger";
}
@Override
public Command getNextAction(World world) {
Command nextAction = ai.getNextAction(world, this);
this.energy--; // Loses 1 energy regardless of action.
return nextAction;
}
@Override
public int getPlantCalories() {
return 0;
}
@Override
public int getStrength() {
return STRENGTH;
}
@Override
public int getViewRange() {
return VIEW_RANGE;
}
@Override
public boolean isDead() {
return energy <= 0;
}
@Override
public void loseEnergy(int energyLoss) {
this.energy = this.energy - energyLoss;
}
@Override
public void moveTo(Location targetLocation) {
location = targetLocation;
}
}
<file_sep>package ca.ubc.ece.cpen221.mp4.ai;
import ca.ubc.ece.cpen221.mp4.Direction;
import ca.ubc.ece.cpen221.mp4.Location;
import ca.ubc.ece.cpen221.mp4.Util;
import ca.ubc.ece.cpen221.mp4.World;
import ca.ubc.ece.cpen221.mp4.commands.Command;
import ca.ubc.ece.cpen221.mp4.commands.DestroyCommand;
import ca.ubc.ece.cpen221.mp4.commands.MoveCommand;
import ca.ubc.ece.cpen221.mp4.commands.WaitCommand;
import ca.ubc.ece.cpen221.mp4.items.Item;
import ca.ubc.ece.cpen221.mp4.vehicles.copy.Vehicle;
/**
* A simple vehicle AI
*/
public class VehicleAI {
protected Vehicle veh;
protected World world;
public VehicleAI() {
}
/**
* Decides the next action to be taken, given the state of the World and the
* vehicle.
*
* @param world
* the current World
* @param animal
* the vehicle waiting for the next action
* @return the next action for vehicle
*/
public Command getNextAction(World world, Vehicle veh) {
this.veh = veh;
this.world = world;
Command com = null;
//vehicle always tries to speed up
veh.speedUp();
//vehicle always maintains direction unless a wall is infront of it
Direction dir = veh.getDirection();
Location targetLocation = new Location(veh.getLocation(), dir);
if (Util.isValidLocation(world, targetLocation) && Util.isLocationEmpty(world, targetLocation)) {
return new MoveCommand(veh, targetLocation);
}
//if the position is not empty the vehicle attempts to run over the object
else{
com = destroyCheck(targetLocation);
}
if (com != null){
return com;
}
//otherwise it must be against a wall so it will turn to a random direction along the wall
//this slows the vehicle down
else {
if (dir == Direction.EAST) {
int temp = (Math.random() <= 0.5) ? 1 : 2;
if (temp == 1 && !veh.getLocation().equals(new Location(world.getWidth(), world.getHeight()))){
dir = Direction.SOUTH;
}
else if(!veh.getLocation().equals(new Location(world.getWidth(),0))) {
dir = Direction.NORTH;
}
else {
dir = Direction.WEST;
}
targetLocation = new Location(veh.getLocation(), dir);
com = destroyCheck(targetLocation);
if (com != null){
return com;
}
if (Util.isValidLocation(world, targetLocation) && Util.isLocationEmpty(world, targetLocation)) {
return new MoveCommand(veh, targetLocation);
}
}
if (dir == Direction.WEST) {
int temp = (Math.random() <= 0.5) ? 1 : 2;
if (temp == 1 && !veh.getLocation().equals(new Location(0, world.getHeight()))){
dir = Direction.SOUTH;
}
else if (!veh.getLocation().equals(new Location(0,0))){
dir = Direction.NORTH;
}
else {
dir = Direction.EAST;
}
targetLocation = new Location(veh.getLocation(), dir);
com = destroyCheck(targetLocation);
if (com != null){
return com;
}
if (Util.isValidLocation(world, targetLocation) && Util.isLocationEmpty(world, targetLocation)) {
return new MoveCommand(veh, targetLocation);
}
}
if (dir == Direction.SOUTH) {
int temp = (Math.random() <= 0.5) ? 1 : 2;
if (temp == 1 && !veh.getLocation().equals(new Location(world.getWidth(), world.getHeight()))){
dir = Direction.EAST;
}
else if (!veh.getLocation().equals(new Location(0, world.getHeight()))) {
dir = Direction.WEST;
}
else {
dir = Direction.NORTH;
}
targetLocation = new Location(veh.getLocation(), dir);
com = destroyCheck(targetLocation);
if (com != null){
return com;
}
if (Util.isValidLocation(world, targetLocation) && Util.isLocationEmpty(world, targetLocation)) {
return new MoveCommand(veh, targetLocation);
}
}
if (dir == Direction.NORTH) {
int temp = (Math.random() <= 0.5) ? 1 : 2;
if (temp == 1 && !veh.getLocation().equals(new Location(world.getWidth(), 0))){
dir = Direction.EAST;
}
else if (!veh.getLocation().equals(new Location(0,0))){
dir = Direction.WEST;
}
else {
dir = Direction.SOUTH;
}
targetLocation = new Location(veh.getLocation(), dir);
com = destroyCheck(targetLocation);
if (com != null){
return com;
}
if (Util.isValidLocation(world, targetLocation) && Util.isLocationEmpty(world, targetLocation)) {
return new MoveCommand(veh, targetLocation);
}
}
}
return veh.stop();
}
/**
* checks whether or not the vehicle is in a collision
*
* @param targetLocation the location directly in front of the vehicle
* @return either a command to destroy the vehicle, the item at the targetLocation or null
*/
public Command destroyCheck(Location targetLocation) {
if (Util.isValidLocation(world, targetLocation) && !Util.isLocationEmpty(world, targetLocation)){
for (Item i : world.getItems()) {
if (i.getLocation().equals(targetLocation)) {
return new DestroyCommand(veh, i);
}
}
}
return null;
}
}<file_sep>package ca.ubc.ece.cpen221.mp4.items.animals;
import javax.swing.ImageIcon;
import ca.ubc.ece.cpen221.mp4.Food;
import ca.ubc.ece.cpen221.mp4.Location;
import ca.ubc.ece.cpen221.mp4.Util;
import ca.ubc.ece.cpen221.mp4.World;
import ca.ubc.ece.cpen221.mp4.ai.AI;
import ca.ubc.ece.cpen221.mp4.ai.ArenaAnimalAI;
import ca.ubc.ece.cpen221.mp4.ai.PredatorAI;
import ca.ubc.ece.cpen221.mp4.commands.Command;
import ca.ubc.ece.cpen221.mp4.items.LivingItem;
/**
* The {@link Bear} is an {@link ArenaAnimal} that uses the {@link PredatorAI}.
*/
public class Bear implements ArenaAnimal {
// Constant attributes of the Bear implementation of ArenaAnimal
private static final int INITIAL_ENERGY = 20;
private static final int MAX_ENERGY = 200;
private static final int STRENGTH = 400;
private static final int VIEW_RANGE = 5;
private static final int MIN_BREEDING_ENERGY = 100;
private static final int COOLDOWN = 5;
private static final int MOVING_RANGE = 1;
private static final ImageIcon bearImage = Util.loadImage("bear.gif");
private final AI ai;
private Location location;
private int energy;
/**
* Constructs a new Bear at a certain initialLocation
*
* @param initialLocation
* the location in which the Bear is created
*/
public Bear(Location initialLocation) {
this.ai = new PredatorAI();
this.location = initialLocation;
this.energy = INITIAL_ENERGY;
}
@Override
public LivingItem breed() {
Bear child = new Bear(location);
child.energy = energy / 2;
this.energy = energy / 2;
return child;
}
@Override
public void eat(Food food) {
energy = Math.min(MAX_ENERGY, energy + food.getMeatCalories());
}
@Override
public int getCoolDownPeriod() {
return COOLDOWN;
}
@Override
public int getEnergy() {
return energy;
}
@Override
public ImageIcon getImage() {
return bearImage;
}
@Override
public Location getLocation() {
return location;
}
@Override
public int getMaxEnergy() {
return MAX_ENERGY;
}
@Override
public int getMeatCalories() {
return energy;
}
@Override
public int getMinimumBreedingEnergy() {
return MIN_BREEDING_ENERGY;
}
@Override
public int getMovingRange() {
return MOVING_RANGE;
}
@Override
public String getName() {
return "Bear";
}
@Override
public Command getNextAction(World world) {
Command nextAction = ai.getNextAction(world, this);
this.energy--;
return nextAction;
}
@Override
public int getPlantCalories() {
return 0;
}
@Override
public int getStrength() {
return STRENGTH;
}
@Override
public int getViewRange() {
return VIEW_RANGE;
}
@Override
public boolean isDead() {
return energy <= 0;
}
@Override
public void loseEnergy(int energyLoss) {
this.energy = this.energy - energyLoss;
}
@Override
public void moveTo(Location targetLocation) {
location = targetLocation;
}
}
| b70884296f89cf80cd94fc3be201c7ae60d073a9 | [
"Java"
] | 6 | Java | tdofher/CPEN221-mp4 | bbaa8290e0c54485d11192bc05d5c224708354b3 | 2ebf8aa89c58cd6eb35c08eb27e0374c153965e9 | |
refs/heads/master | <repo_name>sahil2211/BigDataLabs<file_sep>/spatial index/spatial2.py
from rtree import index
import math
import time
import sys
minimumx = 0
minimumy = 0
maximumx = 0
maximumy = 0
class Point:
def __init__(self,x,y):
self.x = x
self.y = y
class Polygon:
def __init__(self,points):
self.points = points
self.nvert = len(points)
global minimumx
global minimumy
global maximumx
global maximumy
minx = maxx = points[0].x
miny = maxy = points[0].y
for i in range(1,self.nvert):
minx = min(minx,points[i].x)
miny = min(miny,points[i].y)
maxx = max(maxx,points[i].x)
maxy = max(maxy,points[i].y)
minimumx = minx
minimumy = miny
maximumx = maxx
maximumy = maxy
self.bound = (minx,miny,maxx,maxy)
def contains(self,pt):
firstX = self.points[0].x
firstY = self.points[0].y
testx = pt.x
testy = pt.y
c = False
j = 0
i = 1
nvert = self.nvert
while (i < nvert) :
vi = self.points[i]
vj = self.points[j]
if(((vi.y > testy) != (vj.y > testy)) and (testx < (vj.x - vi.x) * (testy - vi.y) / (vj.y - vi.y) + vi.x)):
c = not(c)
if(vi.x == firstX and vi.y == firstY):
i = i + 1
if (i < nvert):
vi = self.points[i];
firstX = vi.x;
firstY = vi.y;
j = i
i = i + 1
return c
def bounds(self):
return self.bound
'''def parseInput():
j = 0
for line in sys.stdin:
line = line.strip()
values = line.split(',')
#if values[0]=='"Date/Time"': continue
if values[0]=="Pickup_latitude": continue
if values[2]=="latitude": continue
yield values
'''
def simplePolygonTest():
print("Point in polygon test")
# Create a simple polygon
JFK = Polygon([Point(40.6188,-73.7712),Point(40.6233,-73.7674),Point(40.6248,-73.7681),Point(40.6281,-73.7681),Point(40.6356,-73.7472),Point(40.6422,-73.7468),Point(40.6469,-73.7534),
Point(40.6460,-73.7544),Point(40.6589,-73.7745),Point(40.6628,-73.7858),Point(40.6634,-73.7891),Point(40.6655,-73.7903),Point(40.6658,-73.8021),Point(40.6632,-73.8146),Point(40.6638,-73.8210),Point(40.6621,-73.8244),Point(40.6546,-73.8248),
Point(40.6469,-73.8212),Point(40.6302,-73.7848),Point(40.6223,-73.7899),Point(40.6203,-73.7831),Point(40.6274,-73.7782),Point(40.6235,-73.7731),Point(40.6193,-73.7738),Point(40.6188,-73.7712)])
LGA = Polygon([Point(40.7662,-73.8888),Point(40.7736,-73.8898),Point(40.7751,-73.8843),Point(40.7808,-73.8852),Point(40.7812,-73.8795),Point(40.7842,-73.8788),Point(40.7827,-73.8751),
Point(40.7864,-73.8711),Point(40.788,-73.8673),Point(40.7832,-73.868),Point(40.7808,-73.8716),Point(40.773,-73.8534),Point(40.7697,-73.8557),Point(40.7673,-73.8505),Point(40.7645,-73.85),Point(40.7637,-73.8529),
Point(40.7676,-73.856),Point(40.7659,-73.8594),Point(40.7654,-73.8625),Point(40.7693,-73.8672),Point(40.7714,-73.8732),Point(40.7697,-73.8871),Point(40.7665,-73.8866),Point(40.7662,-73.8888)])
List_points=[]
JFKcount=0
LGAcount=0
with open("taxigreen(06-15)_table.csv") as f:
for line in f:
line=line.strip()
values=line.split(",")
if values[0]=="Pickup_latitude" or values[0]=="latitude":
continue
dropoff_latitude=float(values[2])
dropoff_longitude=float(values[3])
pt1=Point(dropoff_latitude,dropoff_longitude)
List_points.append(pt1)
start_time = time.clock()
for i1 in range(0,len(List_points)):
if JFK.contains(List_points[i1]):
JFKcount=JFKcount+1
JFK_time = (time.clock() - start_time)
start_time = time.clock()
for i1 in range(0,len(List_points)):
if LGA.contains(List_points[i1]):
LGAcount = LGAcount+1
LGA_time =(time.clock() - start_time)
print("query time for jfk is %f seconds" %(JFK_time))
print("Total points in the polygon of JFK is %d " % (JFKcount))
print("query time for LGA is %f seconds" %(LGA_time))
print("Total points in the polygon of LGA is %d" %(LGAcount))
def simpleRTree():
i=1
idx = index.Index()
JFK = Polygon([Point(40.6188,-73.7712),Point(40.6233,-73.7674),Point(40.6248,-73.7681),Point(40.6281,-73.7681),Point(40.6356,-73.7472),Point(40.6422,-73.7468),Point(40.6469,-73.7534),
Point(40.6460,-73.7544),Point(40.6589,-73.7745),Point(40.6628,-73.7858),Point(40.6634,-73.7891),Point(40.6655,-73.7903),Point(40.6658,-73.8021),Point(40.6632,-73.8146),Point(40.6638,-73.8210),Point(40.6621,-73.8244),Point(40.6546,-73.8248),
Point(40.6469,-73.8212),Point(40.6302,-73.7848),Point(40.6223,-73.7899),Point(40.6203,-73.7831),Point(40.6274,-73.7782),Point(40.6235,-73.7731),Point(40.6193,-73.7738),Point(40.6188,-73.7712)])
with open("taxigreen(06-15)_table.csv") as f:
for line in f:
line=line.strip()
values=line.split(",")
if values[0]=="Pickup_latitude" or values[0]=="latitude":
continue
dropoff_latitude=float(values[2])
dropoff_longitude=float(values[3])
pt1=Point((dropoff_latitude),(dropoff_longitude))
idx.insert(i,(pt1.x,pt1.y,pt1.x,pt1.y))
i=i+1
#print(i)
leftBottom = Point(minimumx,minimumy)
rightTop = Point(maximumx,maximumy)
start_time = time.clock()
results = list(idx.intersection(((leftBottom.x),(leftBottom.y),(rightTop.x),(rightTop.y))))
JFK_time=(time.clock() - start_time)
print("JFK Query result ")
#print((results))
print("query time for jfk is %f seconds " %(JFK_time))
print("Total points in the polygon of JFK is %d " % (len(results)))
LGA = Polygon([Point(40.7662,-73.8888),Point(40.7736,-73.8898),Point(40.7751,-73.8843),Point(40.7808,-73.8852),Point(40.7812,-73.8795),Point(40.7842,-73.8788),Point(40.7827,-73.8751),
Point(40.7864,-73.8711),Point(40.788,-73.8673),Point(40.7832,-73.868),Point(40.7808,-73.8716),Point(40.773,-73.8534),Point(40.7697,-73.8557),Point(40.7673,-73.8505),Point(40.7645,-73.85),Point(40.7637,-73.8529),
Point(40.7676,-73.856),Point(40.7659,-73.8594),Point(40.7654,-73.8625),Point(40.7693,-73.8672),Point(40.7714,-73.8732),Point(40.7697,-73.8871),Point(40.7665,-73.8866),Point(40.7662,-73.8888)])
leftBottom = Point(minimumx,minimumy)
rightTop = Point(maximumx,maximumy)
start_time = time.clock()
results = list(idx.intersection(((leftBottom.x),(leftBottom.y),(rightTop.x),(rightTop.y))))
LGA_time =(time.clock() - start_time)
print("LGA Query result ")
#print((results))
print("query time for LGA is %f seconds" %(LGA_time))
print("Total points in the polygon of LGA is %d" %(len(results)))
simplePolygonTest()
#sys.stdin = sys.__stdin__
simpleRTree()
<file_sep>/spatial index/README.md
Part 1 - Using a database for spatial queries
-------
1. Load the taxi data into MySQL.
2. Run a query to identify all trips whose drop-off points are in JFK. Report the result size and query execution time.
3. Run a query to identify all trips whose drop-off points are in LaGuardia. Report the result size and query execution time.
4. Now, create a spatial index on drop-off points.
5. Re-run the above two queries, and report the result sizes and query execution times.
Part 2 - Using custom code for spatial queries
------
1. Read the taxi data using python.
2. Using the provided functions, identify all trips whose drop-off points are in JFK. Report the result size and query execution time.
This is accomplished by iterating through all trips, and for each trip checking whether the drop-off location is within the query polygon of not.
3. Identify all trips whose drop-off points are in LaGuardia, using the same technique as above. Report the result size and query execution time.
4. Create a R-tree index on drop-off locations using the Rtree module.
5. Execute the above two queries using the Rtree. As mentioned in the lab, the Rtree only supports rectangular queries. So a polygonal query can be executed as follows:
a. Obtain the bound of a given polygon
b. Query on Rtree using this bound
c. For each resulting point, check if that point is within the polygon or not (using a loop, similar to (2) above).
6. Report the result sizes and running times. Briefly discuss why the running times are different between (2,3) and (5).
Deliverable:
Due Monday, May 2, 2016 at 12:00pm (noon).
Submit
1. A text file to NYU Classes that gives your answers to Part I,#2,#3,#5 and Part II,#2,#3,#5,#6.
2. A text file with SQL commands used for Part 1
3. Python code for Part 2.
| 18149602b2b31aee825cef1ad3300d605b37cc5b | [
"Markdown",
"Python"
] | 2 | Python | sahil2211/BigDataLabs | d3782b668474887e0c3c4b467dcd41b91e39c75a | 5b91995401af98d1237c0758204e4f028764a381 | |
refs/heads/master | <repo_name>TingYinHelen/qiankun-demo<file_sep>/app1/src/main.js
import Vue from 'vue';
import ElementUI from 'element-ui';
import 'element-ui/lib/theme-chalk/index.css';
import App from './App.vue';
Vue.config.productionTip = false;
Vue.use(ElementUI);
function render(props = {}) {
const { container } = props;
console.log('container====1', container);
new Vue({
render: (h) => h(App),
}).$mount(container ? container.querySelector('#app') : '#app');
}
export async function bootstrap() {
console.log('[vue] vue app bootstraped');
}
export async function mount(props) {
console.log('[vue] props from main framework', props);
// storeTest(props);
render(props);
}
export async function unmount() {
console.log('[ unmount ]');
// instance.$destroy();
// instance.$el.innerHTML = '';
// instance = null;
// router = null;
}
| 5ff4667b364e2f718ee82fd71d02fadb5cffd1f1 | [
"JavaScript"
] | 1 | JavaScript | TingYinHelen/qiankun-demo | a9d13d4d91b6ecbd702f0f4828da2da4a3e56f40 | f3ed073e1e1f837bf9f25cc96f991d608ca78e0f | |
refs/heads/master | <file_sep># MIT License
#
# Copyright (c) 2017 <NAME>
#
# Permission is hereby granted, free of charge, to any person obtaining a copy
# of this software and associated documentation files (the "Software"), to deal
# in the Software without restriction, including without limitation the rights
# to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
# copies of the Software, and to permit persons to whom the Software is
# furnished to do so, subject to the following conditions:
#
# The above copyright notice and this permission notice shall be included in all
# copies or substantial portions of the Software.
#
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
# IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
# FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
# AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
# LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
# OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
#SOFTWARE.
import hashlib
import hmac
import requests
import time
import urllib
BASE_URL = 'https://bittrex.com/api/v1.1/{}/{}'
class BittrexAPIBind(object):
def __init__(self, api_key=None, api_secret=None):
self.api_key = api_key
self.api_secret = api_secret
def _api_query(self, scope, req, **kwargs):
tokens = ('{}?').format(req)
request_header = None
if scope == 'account':
nonce = str(int(time.time()/100))
tokens += ('apikey={}&nonce={}').format(self.api_key, nonce)
if kwargs:
tokens += ('&{}').format(urllib.urlencode(kwargs))
request_url = (BASE_URL).format(scope, tokens)
if scope == 'account':
api_sign = hmac.new(self.api_secret.encode(),
request_url.encode(),
hashlib.sha512).hexdigest()
request_header = dict(apisign=api_sign)
return requests.get(request_url, headers=request_header).json()
def get_market_summaries(self):
resp = self._api_query('public', 'getmarketsummaries')
return resp['result']
def get_market_summary(self, currency_base, currency_market):
market = ('{}-{}').format(currency_base, currency_market)
resp = self._api_query('public', 'getmarketsummary', market=market)
market = resp['result'][0]
return market
def get_ticker_last(self, currency_base, currency_market):
market = ('{}-{}').format(currency_base, currency_market)
resp = self._api_query('public', 'getticker', market=market)
market = resp['result']['Last']
return market
def get_balances(self):
resp = self._api_query('account', 'getbalances')
cnt = -1
wallet = resp['result']
while cnt < len(wallet)-1:
cnt += 1
if not wallet[cnt].get('Balance') or wallet[cnt].get('Currency') in ["BTC", "ETH"]:
del wallet[cnt]
cnt -= 1
return resp['result']
<file_sep>#!/usr/bin/env python
# flake8: noqa
from setuptools import setup, find_packages
setup(
name = "bit-bind",
version = "0.0.1",
packages = find_packages(),
author = "<NAME>",
author_email = "<EMAIL>",
description = "Bittrex SMS Notifier",
license = "MIT",
keywords = "sms bittrex",
url = "https://github.com/hthompson6/bit-bind",
long_description = open('README.md').read(),
classifiers = [
'Programming Language :: Python',
'Programming Language :: Python :: 2.7',
'Operating System :: OS Independent',
'License :: OSI Approved :: MIT License',
'Development Status :: 3 - Alpha',
]
)
<file_sep># bit_bind
Custom API bindings for bittrex
## Installation
```
$ pip install bit-bind
```
### Install from source
```
$ git clone https://github.com/hthompson6/bit-bind.git
$ cd bit-bind
$ python setup.py install -e .
```
## Usage
```python
api_key = `<KEY>`
api_secret = `zzzzzzzzzzzzzz`
bit_bound = BittrexAPIBinder(api_key, api_secret)
bit_bound.get_current_balances()
bit_bound.get_current_value('BTC', 'VOX')
```
| c1ccbe713c83432525c0d384a94b00f442fd9c89 | [
"Markdown",
"Python"
] | 3 | Python | hthompson-a10/bit-bind | ba7eeb704f9efcebced7e8a4c49700458c4fe987 | 91d0f31b73841550f48413a352e9818761fdb292 | |
refs/heads/master | <file_sep>// export function
exports.getPI = function(value) {
return 3.14;
};<file_sep>var module1 = require('module1');
ctx.pi = module1.getPI(); | a33c204cb632126b34e2fc4177a123272793e7ad | [
"JavaScript"
] | 2 | JavaScript | richardwilly98/elasticsearch-lang-javascript | e3c03936fdd0caa0407ff108aa22d363ce24c2a1 | 9f1b4fc1a1ce129798287a1ff93021c56132da5d | |
refs/heads/main | <file_sep>import pandas as pd
def load_sample():
df = pd.read_csv(
"https://gist.githubusercontent.com/rgbkrk/a7984a8788a73e2afb8fd4b89c8ec6de/raw/db8d1db9f878ed448c3cac3eb3c9c0dc5e80891e/2015.csv"
)
return df.set_index("Country")
<file_sep># dx Design Concepts
## Use cases
- Store a chart view
- Load a stored chart into a notebook
- Save chart settings as a template
- Programmatic creation of a chart
## JSON Schema for data-explorer
Update the current schema files for data-explorer as they currently fail
when trying to convert to a Python model
## Convert JSON Schema to Python model
- Check for valid JSON Schema doc before generating Python code
- Use **datamodel-code-generator** to convert JSON, JSON Schema or OpenAPI to
Python model.
## Store the Python model for programmatic use
## Workflow
- Create a notebook
- Display a chart
- Save the notebook
- Extract the chart cell's metadata
- Create a second notebook for programmatic execution
- Pass the cell's metadata converted to Python code
- Render the chart from the Python code in a notebook
<file_sep>dataclasses-json
datamodel-code-generator
jsonschema
jupyter
marshmallow
nteract_on_jupyter
pandas
pydantic
<file_sep># CONTRIBUTING
We use flit to package this project for PyPI.<file_sep>"""Generate python pydantic models from JSON Schema files."""
from pathlib import Path
from tempfile import TemporaryDirectory
from datamodel_code_generator import InputFileType, generate
# TODO convert to file later
json_schema: str = """{
"$schema": "http://json-schema.org/draft-07/schema#",
"title": "Person",
"type": "object",
"properties": {
"firstName": {
"type": "string",
"description": "The person's first name."
},
"lastName": {
"type": "string",
"description": "The person's last name."
},
"age": {
"description": "Age in years which must be equal to or greater than zero.",
"type": "integer",
"minimum": 0
},
"friends": {
"type": "array"
},
"comment": {
"type": "null"
}
}
}
"""
with TemporaryDirectory() as temporary_directory_name:
temporary_directory = Path(temporary_directory_name)
output = Path(temporary_directory / 'model.py')
generate(
json_schema,
input_file_type=InputFileType.JsonSchema,
input_filename="example.json",
output=output,
)
model: str = output.read_text()
print(model)
<file_sep>black
flit
isort
pytest
tox
<file_sep># dx
[](https://mybinder.org/v2/gh/nteract/dx/main?urlpath=nteract/tree/examples)
A Pythonic Data Explorer.
## Install
For Python 3.8+:
```
pip install dx
```
## Usage
The `dx` library contains a simple helper function also called `dx`.
```python
from dx import dx
```
`dx()` takes one positional argument, a `dataframe`.
```python
dx(dataframe)
```
The `dx(dataframe)` function will display the dataframe in
[data explorer](https://github.com/nteract/data-explorer) mode:
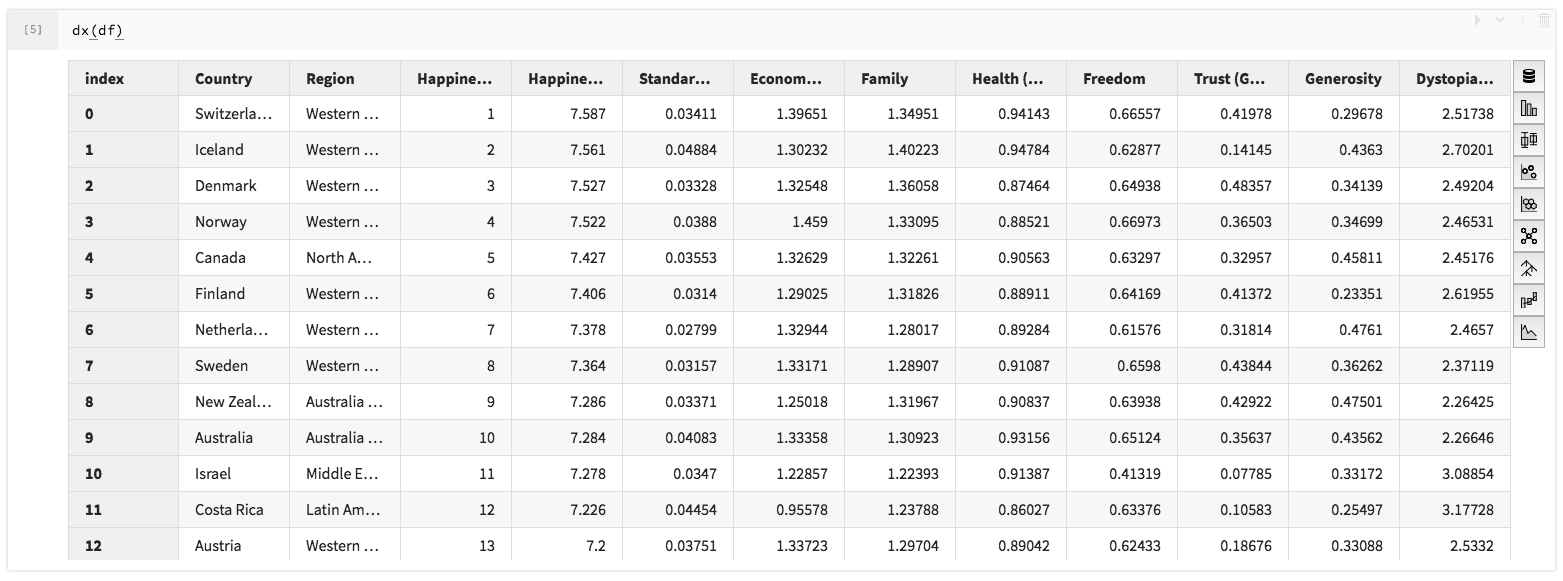
Today, a Pandas `DataFrame` may be passed. In the future, other dataframe types
may be supported.
### Example
```python
import pandas as pd
from dx import dx
# Get happiness data and create a pandas dataframe
df = pd.read_csv('examples/data/2019.csv')
# Open data explorer with the happiness dataframe
dx(df)
```
If you only wish to display a certain number of rows from the dataframe, use
a context and specify the max rows (if set to None, all rows are used):
```python
# To use the first 13 rows for visualization with dx
with pd.option_context('display.max_rows', 13):
dx(df)
```
## FAQ
Q: What about Spark?
A: Spark support would be highly welcome!
See [improved-spark-viz](https://github.com/nteract/improved-spark-viz) for
the current effort. There's a format that pandas handles for us that we could
create in spark land.
## Develop
```
git clone https://github.com/nteract/dx
cd dx
pip install -e .
```
We currently install jupyter and jupyter_on_nteract packages for ease of running
examples.
To run nteract on jupyter:
```
jupyter nteract
```
## Code of Conduct
We follow the nteract.io code of conduct.
## LICENSE
See [LICENSE.md](LICENSE.md).
<file_sep>"""A Pythonic Data Explorer"""
__version__ = '0.3.0.dev0'
import pandas as pd
from .datasets import load_sample
def dx(dataframe, sampled=None):
"""Create dx from a dataframe"""
# All the metadata keys that we'll apply for just the data explorer media
# type, `application/vnd.dataresource+json`
scoped_metadata = {}
if sampled:
scoped_metadata["sampled"] = sampled
metadata = {"application/vnd.dataresource+json": scoped_metadata}
with pd.option_context('display.html.table_schema', True):
display(dataframe, metadata=metadata)
def enable():
"""Enable html table display"""
pd.options.display.html.table_schema = True
def disable():
"""Disable html table display"""
pd.options.display.html.table_schema = False
<file_sep>[build-system]
requires = ["flit_core >=2,<4"]
build-backend = "flit_core.buildapi"
[tool.flit.metadata]
module = "dx"
author = "nteract"
author-email = "<EMAIL>"
home-page = "https://github.com/nteract/dx"
description-file = "README.md"
requires = [
"datamodel-code-generator",
"dataclasses-json",
"jsonschema",
"jupyter",
"marshmallow",
"nteract_on_jupyter",
"pandas",
"pydantic"
]
classifiers = [
"Programming Language :: Python :: 3.8",
"Programming Language :: Python :: 3.9"
]
dist-name = "dx"
[tool.flit.metadata.requires-extra]
dev = [
"black",
"flit",
"isort",
"pre-commit",
"pytest",
"tox"
]
test = [
"pytest >=2.7.3",
"pytest-cov",
]
doc = ["sphinx"]
[tool.black]
line-length = 88
target-version = ['py38']
include = '\.pyi?$'
extend-exclude = '''
# A regex preceded with ^/ will apply only to files and directories
# in the root of the project.
^/foo.py # exclude a file named foo.py in the root of the project (in addition to the defaults)
'''
[tool.isort]
profile = "black"
multi_line_output = 3
<file_sep>[tox]
envlist = testenv, py39
isolated_build = true
[testenv]
basepython = python3.9
command = pytest -vv .
[testenv:py39]
basepython = python3.9
<file_sep>import pytest
def test_load_sample():
pass
def test_load_sample_fails():
pass
| 82328339e6c310046cfce6a7d4eaaed4ee662f6a | [
"Markdown",
"TOML",
"INI",
"Python",
"Text"
] | 11 | Python | nteract/dx | 7a021b9830d844373331341858309b1ba2f60468 | 4109b275d3a0599d627b63989d54b9f2bf69b29f | |
refs/heads/main | <repo_name>shmeeli/hw02-4550<file_sep>/calc.js
(function() {
"use strict";
var displayText = "";
var val1 = null;
var val2 = 0;
var currentArg = 1;
var operation = null;
var zero = document.getElementById("zero");
var one = document.getElementById("one");
var two = document.getElementById("two");
var three = document.getElementById("three");
var four = document.getElementById("four");
var five = document.getElementById("five");
var six = document.getElementById("six");
var seven = document.getElementById("seven");
var eight = document.getElementById("eight");
var nine = document.getElementById("nine");
var plusequals = document.getElementById("plusequals");
var minus = document.getElementById("minus");
var times = document.getElementById("times");
var divide = document.getElementById("divide");
var point = document.getElementById("point");
var clear = document.getElementById("clear");
var display = document.getElementById("screen");
function init() {
zero.addEventListener("click", zerof);
one.addEventListener("click", onef);
two.addEventListener("click", twof);
three.addEventListener("click", threef);
four.addEventListener("click", fourf);
five.addEventListener("click", fivef);
six.addEventListener("click", sixf);
seven.addEventListener("click", sevenf);
eight.addEventListener("click", eightf);
nine.addEventListener("click", ninef);
plusequals.addEventListener("click", plusequalsf);
minus.addEventListener("click", minusf);
times.addEventListener("click", timesf);
divide.addEventListener("click", dividef);
point.addEventListener("click", pointf);
clear.addEventListener("click", clearf);
display.innerText = "";
}
function append_digit(digit) {
if (displayText == "0" || displayText == "Undefined"
|| display.innerText == "Undefined"
|| (currentArg == 1 && val1 != null)) {
displayText = digit;
val1 = null;
} else {
displayText += digit;
}
show();
}
function show() {
if (displayText == "NaN"){
displayText = "Undefined";
}
displayText = (""+displayText).substring(0,16);
display.innerText = displayText;
}
function clearf() {
val1 = null;
displayText = "";
currentArg = 1;
show();
}
function pointf() {
if (!displayText.includes(".")) {
if (displayText == "" || displayText == "Undefined") {
displayText = "0.";
} else if ((currentArg == 1 && val1 == null) || currentArg == 2){
displayText += ".";
}
}
show();
}
function dividef() {
if (currentArg == 1) {
val1 = parseFloat(displayText);
displayText = "";
operation = "divide"
currentArg = 2;
}
show();
}
function timesf() {
if (currentArg == 1) {
val1 = parseFloat(displayText);
displayText = "";
operation = "times"
currentArg = 2;
}
show();
}
function minusf() {
if (currentArg == 1 && displayText != "") {
val1 = parseFloat(displayText);
displayText = "";
operation = "minus"
currentArg = 2;
} else if (displayText == "") {
displayText = "-";
}
show();
}
function plusequalsf() {
if (currentArg == 1) {
val1 = parseFloat(displayText);
displayText = "";
operation = "plus"
currentArg = 2;
} else if (currentArg == 2 && displayText != "") {
if (operation == "plus") {
displayText = "" + (val1 + parseFloat(displayText));
} else if (operation == "minus"){
displayText = "" + (val1 - parseFloat(displayText));
} else if (operation == "times"){
displayText = "" + (val1 * parseFloat(displayText));
} else if (operation == "divide"){
if (parseFloat(displayText) != 0) {
displayText = "" + (val1 / parseFloat(displayText));
} else {
displayText = "Undefined";
currentArg = 1;
val1 = null;
}
}
operation = null;
currentArg = 1;
}
show();
}
function zerof() {
if (displayText == "Undefined" || (currentArg == 1 && val1 != null)){
displayText = "0";
} else if (displayText != "0") {
displayText += "0";
}
show();
}
function onef() {
append_digit(1);
}
function twof() {
append_digit(2);
}
function threef() {
append_digit(3);
}
function fourf() {
append_digit(4);
}
function fivef() {
append_digit(5);
}
function sixf() {
append_digit(6);
}
function sevenf() {
append_digit(7);
}
function eightf() {
append_digit(8);
}
function ninef() {
append_digit(9);
}
window.addEventListener('load', init, false);
})();
<file_sep>/test.js
(function() {
"use strict";
function init() {
var display = document.getElementById("screen");
display.innerText = "hi";
}
window.addEventListener('load', init, false);
})();
| a23263a2de106a217a8f4967c3146f9336b3fffc | [
"JavaScript"
] | 2 | JavaScript | shmeeli/hw02-4550 | 032eb1d0178c84487963c13232dc8d2b924a96a3 | f417e1c23b0080834f41fcf7f0f74012d88b2ee6 | |
refs/heads/master | <file_sep>#def fizz_buzz(number)
# number
#end
# def fizz_buzz(number)
# if number %15 ==0
# 'fizzbuzz'
#elsif number % 5 ==0
# 'buzz'
# elsif number % 3 == 0
# 'fizz'
#else
# number
# end
#end
def fizz_buzz(number)
if has_zero_remainder?(number, 15)
'fizzbuzz'
elsif has_zero_remainder?(number, 5)
'buzz'
elsif has_zero_remainder?(number, 3)
'fizz'
else
number
end
end
def has_zero_remainder?(number, divider)
number % divider == 0
end | 3e26766ba4a93896e0d33085d88c2ad5a797630c | [
"Ruby"
] | 1 | Ruby | raomanasa/fizzbuzz | 0f1c20e071b4b9e7362d18bf983950c9b93fc7c7 | a50c4c833f84ae01f66f7163d94c45995c0b4bdd | |
refs/heads/master | <repo_name>bianliuzhu/componentPack<file_sep>/src/router/index.js
import Vue from 'vue'
import Router from 'vue-router'
Vue.use(Router)
const login = r => require.ensure([], () => r(require('@/page/login')), 'login');
const manage = r => require.ensure([], () => r(require('@/page/manage')), 'manage');
const home = r => require.ensure([], () => r(require('@/page/home')), 'home');
const addShop = r => require.ensure([], () => r(require('@/page/addShop')), 'addShop');
const pagination = r => require.ensure([], () => r(require('@/page/pagination')), 'pagination');
const shearchBox = r => require.ensure([], () => r(require('@/page/searchBox')), 'searchBox');
const table = r => require.ensure([], () => r(require('@/page/table')), 'table');
const formList = r => require.ensure([], () => r(require('@/page/formList')), 'formList');
const gtTabTree = r => require.ensure([], () => r(require('@/page/gtTabTree')), 'gtTabTree');
const dialog = r => require.ensure([], () => r(require('@/page/dialog')), 'dialog');
const upload = r => require.ensure([], () => r(require('@/page/upload')), 'upload');
const newTag = r => require.ensure([], () => r(require('@/page/newTag')), 'newTag');
const autocomplete = r => require.ensure([], () => r(require('@/page/autocomplete')), 'autocomplete');
const userEditor = r => require.ensure([], () => r(require('@/page/userEditor')), 'userEditor');
const editor = r => require.ensure([], () => r(require('@/page/editor')), 'editor');
const cascader = r => require.ensure([], () => r(require('@/page/cascader')), 'cascader');
const brand = r => require.ensure([], () => r(require('@/page/brand')), 'brand');
const goodsPublish = r => require.ensure([], () => r(require('@/page/goodsPublish')), 'goodsPublish');
const userList = r => require.ensure([], () => r(require('@/page/userList')), 'userList');
const routes = [{
path: '/',
component: login
},
{
path: '/manage',
component: manage,
children: [{
path: '',
component: home,
meta: [],
}, {
path: '/addShop',
component: addShop,
meta: ['添加数据', '添加商铺'],
}, {
path: '/pagination',
component: pagination,
meta: ['添加数据', '添加商品'],
}, {
path: '/shearchBox',
component: shearchBox,
meta: ['组件', '搜索框'],
}, {
path: '/table',
component: table,
meta: ['组件', '表格'],
}, {
path: '/formList',
component: formList,
meta: ['组件', 'form表单'],
}, {
path: '/gtTabTree',
component: gtTabTree,
meta: ['组件', '订单列表'],
}, {
path: '/dialog',
component: dialog,
meta: ['数据管理', '管理员列表'],
}, {
path: '/upload',
component: upload,
meta: ['图表', '用户分布'],
}, {
path: '/newTag',
component: newTag,
meta: ['图表', '用户数据'],
}, {
path: '/autocomplete',
component: autocomplete,
meta: ['文本编辑', 'MarkDown'],
}, {
path: '/userEditor',
component: userEditor,
meta: ['编辑', '文本编辑'],
}, {
path: '/userList',
component: userList,
meta: ['编辑', '文本编辑'],
}, {
path: '/editor',
component: editor,
meta: ['设置', '管理员设置'],
}, {
path: '/goodsPublish',
component: goodsPublish,
meta: ['发布', '商品'],
}, {
path: '/brand',
component: brand,
meta: ['说明', '说明'],
}]
}
]
export default new Router({
routes,
strict: process.env.NODE_ENV !== 'production',
})
| 2d051e3842b640b769520cd5c3722f01c8aa0d89 | [
"JavaScript"
] | 1 | JavaScript | bianliuzhu/componentPack | ee4a5d3f5517ac8ed2428110823acba9b4300fb0 | e208bce7dcd239433902f0b9dbc036f0a56031da | |
refs/heads/master | <file_sep>/*
* Name: <NAME>
* Date: May 19, 2020
* Section: CSE 154 AJ
*
* This is the JS to implement the UI
*/
"use strict";
(function() {
window.addEventListener("load", starter);
let gameArray = TEXT;
console.log(TEXT);
let packArray = [true, true, true, true, true, true, true];
/**
* This function is the starter function for everything that I want
* to fire when the HTML page loads
* no parameters, no returns
*/
function starter() {
/**
* what options do I want to add:
* sort/filter by jackbox party pack number
* something that shows player num/range and party pack number in the textbox
*/
id("all-games").innerHTML = "";
for (let i = 0; i < gameArray.length; i++) {
console.log(i);
addGames(i);
}
let enterButton = document.getElementById("enter");
enterButton.addEventListener("click", clickEnter);
let homeButton = document.getElementById("home");
homeButton.addEventListener("click", homeButton);
}
function addAllGames(gameArray, players){
packArray = [id("box_1").checked,id("box_2").checked,id("box_3").checked,id("box_4").checked,id("box_5").checked,id("box_6").checked,id("box_7").checked]
id("all-games").innerHTML = "";
players = parseInt(players);
console.log(players);
for (let i = 0; i < gameArray.length; i++) {
let min = gameArray[i].min;
let max = gameArray[i].max;
if(players >= min && players <= max){
addGames(i);
}
}
}
function addGames(i){
console.log(i);
console.log(packArray[gameArray[i].pack]-1);
if(packArray[gameArray[i].pack-1]){
console.log(i);
let link = document.createElement("a");
let div = document.createElement("div");
let gameName = gameArray[i].gamename;
let text = document.createElement("p");
text.innerHTML = gameName + ":" +"<br>" + gameArray[i].description ;
let range = document.createElement("p");
range.innerHTML = "range: " + gameArray[i].min + "-" + gameArray[i].max + "p";
range.classList.add("ranges");
let pack_num = document.createElement("p");
pack_num.innerHTML = "Party Pack: " + gameArray[i].pack;
pack_num.classList.add("pack_num");
let image = document.createElement("img");
image.src = "images/" + gameArray[i].image + ".jpg";
div.id = gameName;
image.alt = gameName;
image.id = "pic_" + gameName;
link.appendChild(div);
div.appendChild(image);
text.appendChild(pack_num);
text.appendChild(range);
div.appendChild(text);
id("all-games").appendChild(link);
}
}
function clickEnter(){
console.log("player input value:" + id("players").value);
addAllGames(gameArray, id("players").value);
}
/**
* This function is the default id function
* @param {string} id - an id string used by html
* @return {element} element gotten by getElementById
*/
function id(id) {
return document.getElementById(id);
}
})();<file_sep>
//sourced from excel file and
//http://www.convertcsv.com/csv-to-json.htm
const TEXT =
[
{
"pack": 1,
"gamename": "Drawful",
"description": "Hilarious drawing and deception game",
"min": 5,
"max": 8,
"image": "drawful"
},
{
"pack": 1,
"gamename": "Fibbage XL",
"description": "Aim to decieve friends while finding the truth",
"min": 2,
"max": 8,
"image": "fibbageXL"
},
{
"pack": 1,
"gamename": "Lie Swatter",
"description": "Find the truth from the flies and swat it down",
"min": 1,
"max": 100,
"image": "lie-swatter"
},
{
"pack": 1,
"gamename": "Word Spud",
"description": "Form strange word clouds",
"min": 2,
"max": 8,
"image": "word-spud"
},
{
"pack": 1,
"gamename": "You Don't Know Jack",
"description": "A terribly fun trivia game",
"min": 1,
"max": 4,
"image": "you-dont-know-jack"
},
{
"pack": 2,
"gamename": "Bidiots",
"description": "Draw and bid on \"Works of Art\" made by you and friends",
"min": 2,
"max": 6,
"image": "bidiots"
},
{
"pack": 2,
"gamename": "Bomb Corp",
"description": "Cooperative bomb defusal game",
"min": 1,
"max": 4,
"image": "bomb-corp"
},
{
"pack": 2,
"gamename": "Earwax",
"description": "Create an auditory experience to shock your friends",
"min": 3,
"max": 8,
"image": "earwax"
},
{
"pack": 2,
"gamename": "Fibbage 2",
"description": "Aim to decieve friends while finding the truth�AGAIN",
"min": 2,
"max": 8,
"image": "fibbage-2"
},
{
"pack": 2,
"gamename": "Quiplash XL",
"description": "Make your friends laugh enough to pick your answer",
"min": 3,
"max": 8,
"image": "quiplashXL"
},
{
"pack": 3,
"gamename": "Fakin' It",
"description": "find the faker in your group by using social deduction",
"min": 3,
"max": 6,
"image": "fakin-it"
},
{
"pack": 3,
"gamename": "Guesspionage",
"description": "Guess the percentage of strange facts",
"min": 2,
"max": 8,
"image": "guesspionage"
},
{
"pack": 3,
"gamename": "Quiplash 2",
"description": "Quiplash, but with custom episodes and cartoons",
"min": 3,
"max": 8,
"image": "quiplash-2"
},
{
"pack": 3,
"gamename": "<NAME>.",
"description": "Create the best custom T-shirts to amuse your friends",
"min": 3,
"max": 8,
"image": "tee-k-o"
},
{
"pack": 3,
"gamename": "Trivia Murder Party",
"description": "A murderously fun trivia game",
"min": 1,
"max": 8,
"image": "trivia-murder-party"
},
{
"pack": 4,
"gamename": "Bracketeering",
"description": "Have dumb arguments about dumber questions",
"min": 3,
"max": 16,
"image": "bracketeering"
},
{
"pack": 4,
"gamename": "Civic Doodle",
"description": "Commit cooperative graffiti with friends and vote on the best",
"min": 3,
"max": 8,
"image": "civic-doodle"
},
{
"pack": 4,
"gamename": "Fibbage 3",
"description": "Fibbage, but with personalized options for close friends",
"min": 2,
"max": 8,
"image": "fibbage-3"
},
{
"pack": 4,
"gamename": "Monster Seeking Monster",
"description": "Multiplayer dating sim where you're all secret monsters",
"min": 3,
"max": 7,
"image": "monster-seeking-monster"
},
{
"pack": 4,
"gamename": "Survive the Internet",
"description": "Try to make your friends tweet strange things",
"min": 3,
"max": 8,
"image": "survive-the-internet"
},
{
"pack": 5,
"gamename": "Mad Verse City",
"description": "Rap battle your friends for glory and bragging rights",
"min": 3,
"max": 8,
"image": "mad-verse-city"
},
{
"pack": 5,
"gamename": "Patently Stupid",
"description": "convince your friends to invest in your genius startup",
"min": 3,
"max": 8,
"image": "patently-stupid"
},
{
"pack": 5,
"gamename": "Split the Room",
"description": "Try to divide your friends opinions with one word",
"min": 3,
"max": 8,
"image": "split-the-room"
},
{
"pack": 5,
"gamename": "You Don't Know Jack-Full Stream",
"description": "Trivia game run by the corporation BinjPipe",
"min": 1,
"max": 8,
"image": "you-dont-know-jack-full-stream"
},
{
"pack": 5,
"gamename": "<NAME>",
"description": "Space-themed platforming fighting game",
"min": 1,
"max": 6,
"image": "zeeple-dome"
},
{
"pack": 6,
"gamename": "Dictionarium",
"description": "Define strange words and give definitions stranger words",
"min": 3,
"max": 8,
"image": "dictionarium"
},
{
"pack": 6,
"gamename": "<NAME>",
"description": "Strange stand-up to survive on a sinking ship",
"min": 3,
"max": 8,
"image": "joke-boat"
},
{
"pack": 6,
"gamename": "Push The Button",
"description": "Try and find alien infiltrators in your spaceship",
"min": 4,
"max": 10,
"image": "push-the-button"
},
{
"pack": 6,
"gamename": "Role Models",
"description": "Pigeonhole your friends into roles",
"min": 3,
"max": 6,
"image": "role-models"
},
{
"pack": 6,
"gamename": "Trivia Murder Party 2",
"description": "Murderously fun trivia game",
"min": 1,
"max": 8,
"image": "trivia-murder-party-2"
},
{
"pack": 7,
"gamename": "Blather Round",
"description": "Describe your word with a very limited vocabulary",
"min": 2,
"max": 6,
"image": "blather-round"
},
{
"pack": 7,
"gamename": "Champ'd Up",
"description": "Create champions and force them to face off for strange titles",
"min": 3,
"max": 8,
"image": "champd-up"
},
{
"pack": 7,
"gamename": "Devil in the Details",
"description": "Collaborate with your friends to survive devil suburbia",
"min": 3,
"max": 8,
"image": "devil-in-the-details"
},
{
"pack": 7,
"gamename": "Quiplash 3",
"description": "It's quiplash for the 3rd time.",
"min": 3,
"max": 8,
"image": "quiplash-3"
},
{
"pack": 7,
"gamename": "Talking Points",
"description": "Intrigue your friends with an on-the-cuff presentation",
"min": 3,
"max": 8,
"image": "talking-points"
}
]
;<file_sep>## Jackbox Sorter
link: https://adam-nicewarner.github.io/jackbox-sorter/
This is a simple site made to help you out with finding the right Jackbox Games for your group of friends. I noticed that there isn't a good repository of Jackbox Games sorted by player number and rough summary, so I made this. If there is anything you'd like to add, please message me on twitter @AdamNicewarner, and I'll do my best to fix it.
| fec51b4df081350d62963974b0e5f3d6fa2f1bbf | [
"JavaScript",
"Markdown"
] | 3 | JavaScript | Adam-Nicewarner/jackbox-sorter | 0b2c65e89288fc8c8c177979d753acfe364f6587 | ce2eae4949e139e5970f1085783a350057fb32c6 | |
refs/heads/master | <repo_name>AKSHARA-T-S-B/javaProject<file_sep>/CyclePriceCalculator/Model/Components/HandleBar/HandleBar.java
package Model.Components.HandleBar;
public class HandleBar {
private PursuitBar[] pursuitBar;
private DropBar[] dropBar;
private RiserBar[] riserBar;
public synchronized PursuitBar[] getPursuitBar() {
return pursuitBar;
}
public synchronized void setPursuitBar(PursuitBar[] pursuitBar) {
this.pursuitBar = pursuitBar;
}
public synchronized DropBar[] getDropBar() {
return dropBar;
}
public synchronized void setDropBar(DropBar[] dropBar) {
this.dropBar = dropBar;
}
public synchronized RiserBar[] getRiserBar() {
return riserBar;
}
public synchronized void setRiserBar(RiserBar[] riserBar) {
this.riserBar = riserBar;
}
}
<file_sep>/Family Tree/FamilyTree.java
package family;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.util.ArrayList;
import java.util.List;
import java.util.Scanner;
import java.util.stream.Collectors;
/**
* Family tree problem
* @author Akshara
*/
class RelationHandler{
/**
* Build default family tree
* @return head of the family
*/
Person buildShanFamily(){
Person root = new Person("Shan", Gender.MALE);
root.addMarriage(new Person("Anga", Gender.FEMALE));
root.addSon("Chit");
root.addSon("Ish");
root.addSon("Vich");
root.addSon("Aras");
root.addDaughter("Satya");
Person chit = searchMember(root, "Chit");
chit.addMarriage(new Person("Amba", Gender.FEMALE));
chit.addDaughter("Dritha");
chit.addDaughter("Tritha");
chit.addSon("Vritha");
Person dritha = searchMember(root, "Dritha");
dritha.addMarriage(new Person("Jaya", Gender.MALE));
dritha.addSon("Yodhan");
Person vich = searchMember(root, "Vich");
vich.addMarriage(new Person("Lika", Gender.FEMALE));
vich.addDaughter("Vila");
vich.addDaughter("Chika");
Person aras = searchMember(root, "Aras");
aras.addMarriage(new Person("Chitra", Gender.FEMALE));
aras.addDaughter("Jnki");
aras.addSon("Ahit");
Person jnki = searchMember(root, "Jnki");
jnki.addMarriage(new Person("Arit", Gender.MALE));
jnki.addSon("Laki");
jnki.addDaughter("Lavnya");
Person satya = searchMember(root, "Satya");
satya.addMarriage(new Person("Vyan", Gender.MALE));
satya.addSon("Asva");
satya.addSon("Vyas");
satya.addDaughter("Atya");
Person asva = searchMember(root, "Asva");
asva.addMarriage(new Person("Satvy", Gender.FEMALE));
asva.addSon("Vasa");
Person vyas = searchMember(root, "Vyas");
vyas.addMarriage(new Person("Krpi", Gender.FEMALE));
vyas.addSon("Kriya");
vyas.addDaughter("Krith");
return root;
}
/**
* Search if the person is part of the family
* @param head
* @param name
* @return the searched person
*/
Person searchMember(Person head, String name) {
if (head == null || head.getName().equalsIgnoreCase(name))
return head;
if(head.getSpouse() != null && head.getSpouse().getName().equalsIgnoreCase(name))
return head.getSpouse();
if (head.getChildren() != null) {
Person temp = null;
for (Person person : head.getChildren()) {
temp = searchMember(person, name);
if (temp != null)
return temp;
}
}
return null;
}
/**
* Add a child to a family
* @param motherName
* @param child
* @param gender
* @param root
*/
public void addMember(String motherName, String child, String gender, Person root) {
Person person = searchMember(root, child);
if(person == null) {
Person mother = searchMember(root, motherName);
if(mother == null)
System.out.println("PERSON_NOT_FOUND");
else if(mother != null && mother.getGender().equals(Gender.FEMALE)) {
Person spouse = mother.getSpouse();
if(spouse == null)
System.out.println("CHILD_ADDITION_FAILED");
else {
mother.addChild(child, gender.equals("Male") ? Gender.MALE : Gender.FEMALE);
System.out.println("CHILD_ADDITION_SUCCEEDED");
}
}else
System.out.println("CHILD_ADDITION_FAILED");
}else
System.out.println("Duplicate person found. CHILD_ADDITION_FAILED");
}
/**
* Print list of persons
* @param personList
*/
public void printList(List<Person> personList) {
if(personList.isEmpty())
System.out.println("NONE");
else {
personList.forEach(action -> {
System.out.print(action.getName() + " ");
});
System.out.println();
}
}
/**
* Print the kids of the mother
* @param person : mother's details
* @param gender : Gender of the kid
*/
public List<Person> getKids(Person person, Gender gender) {
List<Person> kidsList = new ArrayList<>();
List<Person> childrenList = person.getChildren();
if(!childrenList.isEmpty())
kidsList.addAll(childrenList.stream()
.filter(action->action.getGender().equals(gender))
.collect(Collectors.toList()));
return kidsList;
}
/**
* Print the maternal or paternal aunts or uncles
* @param parent
* @param gender
*/
public List<Person> getAuntUncle(Person parent, Gender gender) {
List<Person> auntUncleList = new ArrayList<>();
List<Person> siblingsList = parent.getSiblings();
if(!siblingsList.isEmpty())
auntUncleList.addAll(siblingsList.stream()
.filter(action->action.getGender().equals(gender))
.collect(Collectors.toList()));
return auntUncleList;
}
/**
* Print the sister-in-law or brother-in-law of the person
* @param person
* @param gender
*/
public List<Person> getInLaws(Person person, Gender gender) {
List<Person> inLawList = new ArrayList<>();
Person spouse = person.getSpouse();
if(spouse != null) {
List<Person> siblingsList = spouse.getSiblings();
if(!siblingsList.isEmpty())
inLawList.addAll(siblingsList.stream()
.filter(action -> action.getGender().equals(gender))
.collect(Collectors.toList()));
}
List<Person> siblings = person.getSiblings();
if(!siblings.isEmpty()) {
List<Person> spouseList = new ArrayList<>();
spouseList.addAll(siblings.stream()
.map(action -> action.getSpouse())
.filter(result -> result != null)
.collect(Collectors.toList()));
inLawList.addAll(spouseList.stream()
.filter(action -> action.getGender().equals(gender))
.collect(Collectors.toList()));
}
return inLawList;
}
/**
* Decode the relationship and invoke the corresponding relation function call
* @param name
* @param relation
* @param root
*/
public void getRelation(String name, String relation, Person root) {
Person person = searchMember(root, name);
if(person == null)
System.out.println("PERSON_NOT_FOUND");
else {
if(relation.equalsIgnoreCase("son"))
printList(getKids(person, Gender.MALE));
if(relation.equalsIgnoreCase("daughter"))
printList(getKids(person, Gender.FEMALE));
if(relation.equalsIgnoreCase("siblings"))
printList(person.getSiblings());;
if(relation.equalsIgnoreCase("paternal-uncle"))
printList(getAuntUncle(person.getFather(), Gender.MALE));
if(relation.equalsIgnoreCase("paternal-aunt"))
printList(getAuntUncle(person.getFather(), Gender.FEMALE));
if(relation.equalsIgnoreCase("maternal-uncle"))
printList(getAuntUncle(person.getMother(), Gender.MALE));
if(relation.equalsIgnoreCase("maternal-aunt"))
printList(getAuntUncle(person.getMother(), Gender.FEMALE));
if(relation.equalsIgnoreCase("sister-in-law"))
printList(getInLaws(person, Gender.FEMALE));
if(relation.equalsIgnoreCase("brother-in-law"))
printList(getInLaws(person, Gender.MALE));
}
}
}
/**
* @author Akshara
* Main program to run the Family tree application
*/
public class FamilyTree {
@SuppressWarnings("resource")
public static void main(String[] args) {
boolean flag = false;
RelationHandler relationHandler = new RelationHandler();
Person root = relationHandler.buildShanFamily();
System.out.println("Enter the location for the test file");
Scanner scanner = new Scanner(System.in);
String filePath = scanner.nextLine();
try {
FileInputStream fileReader = new FileInputStream(filePath);
scanner = new Scanner(fileReader);
while(scanner.hasNextLine()) {
String input = scanner.nextLine();
String[] splitString = input.split("\\s+");
if(splitString.length > 0 && splitString[0].contains("ADD_CHILD")) {
flag = true;
if(splitString.length != 4)
System.out.println(input.trim() + " - Insufficient details provided to add a child");
else {
relationHandler.addMember(splitString[1], splitString[2], splitString[3], root);
}
}
if(splitString.length > 0 && splitString[0].contains("GET_RELATIONSHIP")) {
flag = true;
if(splitString.length != 3)
System.out.println(input + " - Insufficient details provided to get a relationship");
else {
relationHandler.getRelation(splitString[1], splitString[2], root);
}
}
}
if(!flag) {
System.out.println("Input file is empty!!!");
}
}catch(FileNotFoundException exception) {
System.out.println("File not found. Incorrect file path specified !!! Try again with correct file path...");
}
}
}
<file_sep>/Car Parking/ParkingAllocation.java
import java.io.*;
import java.util.*;
import java.util.stream.Collectors;
/**
* Car parking allocation problem
* @author Akshara
*
*/
class Car{
String regNo;
String colour;
Car(){
this.regNo = null;
this.colour = null;
}
Car(String regNo, String colour){
this.regNo = regNo;
this.colour = colour;
}
String getRegNo(){
return regNo;
}
String getColour(){
return colour;
}
boolean isNull(){
if(this.regNo == null && this.colour == null){
return true;
}
return false;
}
}
class ParkingSpace{
//createParkingSlot is to create a parking slot based on user defined input
int createParkingSlot() throws IOException{
int parkingSlot = 0;
System.out.println("Enter the number of slots to create a parking lot");
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
try{
parkingSlot = Integer.parseInt(reader.readLine());
}catch(NumberFormatException exception){
}
return parkingSlot;
}
//parkVehicles will allocate the available slots for the vehicles which needed to be parked
Map<String, Object> parkVehicles(TreeMap<Integer, Car> parkingMap, Car car){
Map<String, Object> resultMap = new HashMap<>();
if(parkingMap.isEmpty()){
parkingMap.put(1, car);
resultMap.put("AllocatedSlot", 1);
}else{
List<Integer> availableSlotList = parkingMap.entrySet()
.stream()
.filter(record -> record.getValue().isNull())
.map(Map.Entry::getKey)
.collect(Collectors.toList());
if(availableSlotList.isEmpty()){
int lastFilledSlot = parkingMap.lastKey();
parkingMap.put(++lastFilledSlot, car);
resultMap.put("AllocatedSlot", lastFilledSlot);
}else{
int slot = availableSlotList.get(0);
parkingMap.put(slot, car);
resultMap.put("AllocatedSlot", slot);
}
}
resultMap.put("ParkingMap", parkingMap);
return resultMap;
}
//leave will depart the parked vehicles
TreeMap<Integer, Car> leave(TreeMap<Integer, Car> parkingMap, int slot){
if(parkingMap.containsKey(slot)){
if(parkingMap.size() > 1){
parkingMap.put(slot, new Car());
}else{
parkingMap = new TreeMap<>();
}
System.out.println("Slot number " + slot + " is free");
}else{
System.out.println("Slot number " + slot + " is already free");
}
return parkingMap;
}
//status is to show the available and filled slots in the parking
void status(TreeMap<Integer, Car> parkingMap){
System.out.print("\nSlot No.\tRegistration No.\tColour\n");
parkingMap.entrySet()
.stream()
.forEach(record -> {
if(!record.getValue().isNull()){
System.out.print(record.getKey() + "\t\t"
+ record.getValue().getRegNo() + "\t\t\t"
+ record.getValue().getColour() + "\n");
}});
}
//findRegistrationNumber will return the registration numbers of the cars based on the colour specified by the user
void findRegistrationNumber(TreeMap<Integer, Car> parkingMap, String colour){
List<String> regNoList = parkingMap.entrySet()
.stream()
.filter(record -> {
if(!record.getValue().isNull() && colour.equals(record.getValue().getColour())){
return true;
}
return false;
})
.map(p -> p.getValue().getRegNo())
.collect(Collectors.toList());
if(regNoList.isEmpty()){
System.out.println("Not found");
}else{
System.out.println("Register numbers for the cars are :");
regNoList.forEach(System.out::println);
}
}
//findSlotNumber will return the slot numbers of the parked cars based on the colour specified by the user
void findSlotNumber(TreeMap<Integer, Car> parkingMap, String colour){
List<Integer> slotNoList = parkingMap.entrySet()
.stream()
.filter(record -> {
if(!record.getValue().isNull() && colour.equals(record.getValue().getColour())){
return true;
}
return false;
})
.map(value -> value.getKey())
.collect(Collectors.toList());
if(slotNoList.isEmpty()){
System.out.println("Not found");
}else{
System.out.println("Slot numbers for the cars are :");
slotNoList.forEach(System.out::println);
}
}
//findByRegistrationNumber will return the slot number for the particular registration number of the car
void findByRegistrationNumber(TreeMap<Integer, Car> parkingMap, String registrationNumber){
int slot = 0;
for (Map.Entry<Integer, Car> slotDetails : parkingMap.entrySet()) {
if(!slotDetails.getValue().isNull()){
if(registrationNumber.equals(slotDetails.getValue().getRegNo())){
slot = slotDetails.getKey();
break;
}
}
}
if(slot == 0){
System.out.println("Not found");
}else{
System.out.println("Slot number : " + slot);
}
}
//findRegNoDuplicate is to find whether the car with the registration number is already parked
boolean findRegNoDuplicate(TreeMap<Integer, Car> parkingMap, String regNo){
if(parkingMap.isEmpty()){
return true;
}
List<Integer> slotList = parkingMap.entrySet().stream()
.filter(record -> {
if(!record.getValue().isNull() && regNo.equals(record.getValue().getRegNo())){
return true;
}
return false;
})
.map(value -> value.getKey())
.collect(Collectors.toList());
if(slotList.isEmpty()){
return true;
}
return false;
}
}
public class ParkingAllocation{
public static void main(String []args) throws IOException{
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
Scanner scanner = new Scanner(System.in);
int parkingSlot, slot, availableSlotFlag;
String regNo, colour;
Map<String, Object> resultMap = new HashMap<>();
TreeMap<Integer, Car> parkingMap = new TreeMap<>();
ParkingSpace parkingSpace = new ParkingSpace();
parkingSlot = parkingSpace.createParkingSlot();
while(parkingSlot <= 0){
System.out.println("Enter the valid number for parking slots. Slots should be greater than 0");
parkingSlot = parkingSpace.createParkingSlot();
}
System.out.println("Created a parking lot with " + parkingSlot + " slots");
availableSlotFlag = parkingSlot;
int operation = 0;
while(true){
System.out.println("\n1 Park vehicles \n2 Leave \n3 Status \n4 Find \n\nNumber entered greater than 4 will exit the program\nEnter the operation to be performed");
try{
operation = Integer.parseInt(reader.readLine());
}catch(NumberFormatException exception){
operation = 0;
}
switch(operation){
case 0 :System.out.println("Enter valid operation");
break;
case 1 ://PARK VEHICLES
if(availableSlotFlag > 0){
System.out.println("Enter the registration number for car");
regNo = scanner.next();
boolean duplicateRegNo = parkingSpace.findRegNoDuplicate(parkingMap, regNo);
if(duplicateRegNo){
System.out.println("Enter the colour of the car");
colour = scanner.next();
Car car = new Car(regNo, colour);
resultMap = parkingSpace.parkVehicles(parkingMap, car);
availableSlotFlag = --availableSlotFlag;
parkingMap = (TreeMap<Integer, Car>)resultMap.get("ParkingMap");
System.out.println("Allocated slot number : " + (int)resultMap.get("AllocatedSlot"));
}else{
System.out.println("There is a car parked already with this register number " + regNo);
}
}else{
System.out.println("Sorry, parking lot is full");
}
break;
case 2 ://LEAVE
System.out.println("Enter the slot number of the vehicle to depart");
try{
slot = Integer.parseInt(reader.readLine());
}catch(NumberFormatException exception){
slot = 0;
System.out.println("Enter valid slot");
}
if(slot > parkingSlot | slot <= 0){
System.out.println("There are totally " + parkingSlot
+ " slot/slots avaiable and slot number starts from 1. So enter valid slot");
}else{
parkingMap = parkingSpace.leave(parkingMap, slot);
++availableSlotFlag;
}
break;
case 3 ://STATUS
System.out.println("There are " + parkingSlot + " parking slot/slots in total");
if(parkingMap.isEmpty()){
System.out.print("All slots are free.");
}else{
parkingSpace.status(parkingMap);
}
break;
case 4 ://FIND
System.out.println("\n1 Find the registration numbers for the cars based on colour \n"
+ "2 Find the slot numbers for the cars based on colour \n"
+ "3 Find slot number for the registration number \nEnter the operation to be performed");
int findOption = 0;
try{
findOption = Integer.parseInt(reader.readLine());
}catch(NumberFormatException exception){
}
switch(findOption){
case 1 ://FIND ALL REG NOs BASED ON CAR COLOUR
System.out.println("Enter the colour");
colour = scanner.next();
if(parkingMap.isEmpty()){
System.out.println("Not Found");
}else{
parkingSpace.findRegistrationNumber(parkingMap, colour);
}
break;
case 2 ://FIND ALL SLOT NOs BASED ON CAR COLOUR
System.out.println("Enter the colour");
colour = scanner.next();
if(parkingMap.isEmpty()){
System.out.println("Not Found");
}else{
parkingSpace.findSlotNumber(parkingMap, colour);
}
break;
case 3 ://FIND THE SLOT NO BASED ON CAR REG NO
System.out.println("Enter the registration number");
regNo = scanner.next();
if(parkingMap.isEmpty()){
System.out.println("Not Found");
}else{
parkingSpace.findByRegistrationNumber(parkingMap, regNo);
}
break;
default: System.out.println("Enter valid option");
}
break;
default:System.exit(0);
}
}
}
}<file_sep>/Family Tree/Family.java
package family;
import java.util.List;
/**
* Model to store the family details of the person
* @author Akshara
*/
public class Family {
private Person husband;
private Person wife;
private List<Person> children;
public Family(Person member1, Person member2) {
super();
this.husband = (member1.getGender().equals(Gender.MALE))?member1:member2;
this.wife = (member1.getGender().equals(Gender.FEMALE))?member1:member2;;
}
public synchronized Person getHusband() {
return husband;
}
public synchronized void setHusband(Person husband) {
this.husband = husband;
}
public synchronized Person getWife() {
return wife;
}
public synchronized void setWife(Person wife) {
this.wife = wife;
}
public synchronized List<Person> getChildren() {
return children;
}
public synchronized void setChildren(List<Person> children) {
this.children = children;
}
}
<file_sep>/CyclePriceCalculator/Model/Components/ChainAssembly/ChainAssembly.java
package Model.Components.ChainAssembly;
public class ChainAssembly {
private FixedGear[] fixedGear;
private Gear3[] gear3;
private Gear4[] gear4;
private Gear5[] gear5;
private Gear6[] gear6;
private Gear7[] gear7;
private Gear8[] gear8;
public synchronized FixedGear[] getFixedGear() {
return fixedGear;
}
public synchronized void setFixedGear(FixedGear[] fixedGear) {
this.fixedGear = fixedGear;
}
public synchronized Gear3[] getGear3() {
return gear3;
}
public synchronized void setGear3(Gear3[] gear3) {
this.gear3 = gear3;
}
public synchronized Gear4[] getGear4() {
return gear4;
}
public synchronized void setGear4(Gear4[] gear4) {
this.gear4 = gear4;
}
public synchronized Gear5[] getGear5() {
return gear5;
}
public synchronized void setGear5(Gear5[] gear5) {
this.gear5 = gear5;
}
public synchronized Gear6[] getGear6() {
return gear6;
}
public synchronized void setGear6(Gear6[] gear6) {
this.gear6 = gear6;
}
public synchronized Gear7[] getGear7() {
return gear7;
}
public synchronized void setGear7(Gear7[] gear7) {
this.gear7 = gear7;
}
public synchronized Gear8[] getGear8() {
return gear8;
}
public synchronized void setGear8(Gear8[] gear8) {
this.gear8 = gear8;
}
}
<file_sep>/Family Tree/Person.java
package family;
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
/**
* Model to store the details of a person and his/her family
* @author Akshara
*
*/
public class Person {
private String name;
private Gender Gender;
private Family family;
private Family parentFamily;
public synchronized Family getFamily() {
return family;
}
public synchronized Family getParentFamily() {
return parentFamily;
}
public Person(String name, Gender Gender){
this.name = name;
this.Gender = Gender;
}
public Person(String name, Gender Gender, Family parentFamily) {
this.name = name;
this.Gender = Gender;
this.parentFamily = parentFamily;
}
public synchronized String getName() {
return name;
}
public synchronized void setName(String name) {
this.name = name;
}
public synchronized Gender getGender() {
return Gender;
}
public synchronized void setGender(Gender Gender) {
this.Gender = Gender;
}
/**
* To get the person's father
* @return the reference of the father
*/
public Person getFather()
{
return (this.parentFamily != null)?this.parentFamily.getHusband():null;
}
/**
* To get the person's mother
* @return the reference of the mother
*/
public Person getMother()
{
return (this.parentFamily != null)?this.parentFamily.getWife():null;
}
/**
* To get the eprson's spouse
* @return the refrence of the spouse
*/
public Person getSpouse()
{
if(this.family != null)
{
return (this.Gender.equals(Gender.MALE))?this.family.getWife():this.family.getHusband();
}
return null;
}
/**
* To get the siblings
* @return the list of siblings
*/
public List<Person> getSiblings()
{
List<Person> result = new ArrayList<>();
if (this.parentFamily != null && this.parentFamily.getChildren() != null) {
result.addAll(this.parentFamily.getChildren().stream().filter(child -> (!child.getName().equalsIgnoreCase(this.getName())))
.collect(Collectors.toList()));
}
return result;
}
public Person addSon(String name)
{
addChild(name, Gender.MALE);
return this;
}
public Person addDaughter(String name)
{
addChild(name, Gender.FEMALE);
return this;
}
/**
* To add a child to a family
* @param childName
* @param Gender
* @return the refernce of a child
*/
public Person addChild(String childName, Gender Gender)
{
if(this.family == null)
System.out.println("There is no such family!!!");
Person child = null;;
if(childName != null && !childName.trim().isEmpty() && this.family != null)
{
child = new Person(childName, Gender, this.family);
if(this.family.getChildren() == null)
this.family.setChildren(new ArrayList<>());
this.family.getChildren().add(child);
}
return child;
}
public List<Person> getChildren()
{
List<Person> result = new ArrayList<>();
if(this.family != null && this.family.getChildren() != null)
result.addAll(this.family.getChildren());
return result;
}
/**
* To add husband or wife to the member of family
* @param spouse : Bride/Groom
* @return : reference Groom/Bride
*/
public Person addMarriage(Person spouse)
{
if(spouse != null)
{
this.family = new Family(this, spouse);
spouse.family = this.family;
return spouse;
}
return null;
}
/**
* print members of the family
* @param root
* @param level
*/
public void printMembers(Person root,int level)
{
if (root == null)
return;
String indent="";
for(int i=level; i > 0; i--)
indent +="\t";
if(root.getSpouse() != null)
System.out.println(indent+"->| "+root.getName()+"/"+root.getSpouse().getName());
else
System.out.println(indent+"->| "+root.getName());
if (root.getChildren() != null) {
level++;
for (Person person : root.getChildren()) {
printMembers(person,level);
}
}
}
}
<file_sep>/CyclePriceCalculator/CyclePricing/TestCycleEstimator.java
package CyclePricing;
import static org.junit.Assert.assertEquals;
import static org.mockito.Matchers.anyInt;
import static org.mockito.Matchers.anyMap;
import static org.mockito.Matchers.anyString;
import java.util.HashMap;
import java.util.Map;
import java.util.concurrent.ArrayBlockingQueue;
import java.util.concurrent.BlockingQueue;
import org.junit.Test;
import org.mockito.Mockito;
import Model.Cycle;
import Model.Components.ChainAssembly.ChainAssembly;
import Model.Components.ChainAssembly.FixedGear;
import Model.Components.ChainAssembly.Gear3;
import Model.Components.ChainAssembly.Gear4;
import Model.Components.ChainAssembly.Gear5;
import Model.Components.ChainAssembly.Gear6;
import Model.Components.ChainAssembly.Gear7;
import Model.Components.ChainAssembly.Gear8;
import Model.Components.Frame.Aluminium;
import Model.Components.Frame.Contilever;
import Model.Components.Frame.Diamond;
import Model.Components.Frame.Frame;
import Model.Components.Frame.Recumbent;
import Model.Components.Frame.Steel;
import Model.Components.Frame.StepThrough;
import Model.Components.Frame.Titanium;
import Model.Components.HandleBar.DropBar;
import Model.Components.HandleBar.HandleBar;
import Model.Components.HandleBar.PursuitBar;
import Model.Components.HandleBar.RiserBar;
import Model.Components.Seating.ComfortSaddle;
import Model.Components.Seating.CruiserSaddle;
import Model.Components.Seating.RacingSaddle;
import Model.Components.Seating.Seating;
import Model.Components.Wheels.Rim;
import Model.Components.Wheels.Spokes;
import Model.Components.Wheels.Tube;
import Model.Components.Wheels.Tyre;
import Model.Components.Wheels.Wheels;
/**
*
* @author AKSHARA
*
*/
public class TestCycleEstimator {
CycleEstimator cycleEstimator = new CycleEstimator();
Cycle cycle = new Cycle();
BlockingQueue<Map<String, Object>> blockingQueue = new ArrayBlockingQueue<>(10);
CyclePriceCalculator cyclePriceCalculator = new CyclePriceCalculator(cycle, blockingQueue);
CyclePriceCalculator cyclePriceCalculatorSpy;
Map<String, Integer> setCostMap() {
Map<String, Integer> costMap = new HashMap<>();
costMap.put("year", 2020);
costMap.put("jan", 250);
costMap.put("feb", 250);
costMap.put("mar", 250);
costMap.put("apr", 260);
costMap.put("may", 260);
costMap.put("jun", 260);
costMap.put("jul", 260);
costMap.put("aug", 270);
costMap.put("sep", 270);
costMap.put("oct", 280);
costMap.put("nov", 280);
costMap.put("dec", 280);
return costMap;
}
/**
* testcyclePriceCalculator is to test cyclePriceCalculator
*/
@Test
public void testcyclePriceCalculator() {
Map<String, String> cyclePriceMap = new HashMap<>();
cyclePriceMap.put("frame", "1200");
cyclePriceMap.put("seating", "580");
cyclePriceMap.put("wheels", "895");
cyclePriceMap.put("chainAssembly", "800");
cyclePriceMap.put("handleBar", "620");
String expected = "4095";
String actual = cyclePriceCalculator.cyclePriceCalculator(cyclePriceMap);
assertEquals(expected, actual);
}
/**
* testGetCurrentYear is to test getCurrentYear()
*/
@Test
public void testGetCurrentYear() {
String expected = "2020";
String actual = cyclePriceCalculator.getCurrentYear();
assertEquals(expected, actual);
}
/**
* testGetCurrentMonth is to test getCurrentMonth
*/
@Test
public void testGetCurrentMonth() {
String expected = "jul";
String actual = cyclePriceCalculator.getCurrentMonth();
assertEquals(expected, actual);
}
/**
* testCycleBuilder is to test cycleBuilder
*/
@Test
public void testCycleBuilder() {
Map<String, Object> cycleMap = new HashMap<>();
cycleMap.put("name", "cycle");
Map<String, String> dateMap = new HashMap<>();
dateMap.put("year", "2020");
dateMap.put("month", "jul");
cycleMap.put("date_of_pricing", dateMap);
cycleMap.put("frame", "diamond, steel");
cycleMap.put("wheels", "");
cycleMap.put("chainAssembly", "fixedGear");
cycleMap.put("seating", "comfort");
cycleMap.put("handleBar", "pursuit");
Map<String, Object> cycleMap1 = new HashMap<>();
cycleMap1.put("name", "cycle");
cycleMap.put("wheels", "");
assertEquals(cycleMap.toString(), cyclePriceCalculator.cycleBuilder(cycleMap1).toString());
}
/**
* testInitialiseSubComponentMap is to test initialiseSubComponentMap
*/
@Test
public void testInitialiseSubComponentMap() {
Map<String, Integer> subComponentMap = new HashMap<>();
subComponentMap.put("rate", 0);
subComponentMap.put("yearFlag", 0);
subComponentMap.put("currentIndex", 0);
assertEquals(subComponentMap, cyclePriceCalculator.initialiseSubComponentMap(new HashMap<String, Integer>()));
}
/**
* testFindRate is to test findRate
*/
@Test
public void testFindRate() {
Map<String, Integer> subComponentMap = new HashMap<>();
subComponentMap.put("rate", 250);
subComponentMap.put("yearFlag", 1);
subComponentMap.put("currentIndex", 0);
subComponentMap.put("year", 2020);
Map<String, Integer> subComponentMap1 = new HashMap<>();
subComponentMap1.put("year", 2020);
Map<String, Integer> costMap = new HashMap<>();
costMap.put("jul", 250);
Map<String, Integer> subComponentMap2 = cyclePriceCalculator.findRate(subComponentMap1, "2020", "jul", costMap,
0);
assertEquals(subComponentMap, subComponentMap2);
}
/**
* testFrameRateCalculator1 is to test frame type diamond in frameRateCalculator
*/
@SuppressWarnings("unchecked")
@Test
public void testFrameRateCalculator1() {
String expected = "520";
Frame frame = new Frame();
Diamond[] diamond = new Diamond[1];
diamond[0] = new Diamond();
diamond[0].setYear(2020);
diamond[0].setCostMap(setCostMap());
frame.setDiamond(diamond);
Steel[] steel = new Steel[1];
steel[0] = new Steel();
steel[0].setYear(2020);
steel[0].setCostMap(setCostMap());
frame.setSteel(steel);
cycle.setFrame(frame);
Map<String, Integer> subComponentMap = new HashMap<>();
subComponentMap.put("yearFlag", 1);
subComponentMap.put("rate", 520);
subComponentMap.put("currentIndex", 0);
subComponentMap.put("year", 2020);
CyclePriceCalculator cyclePriceCalculator1 = new CyclePriceCalculator(cycle, blockingQueue);
CyclePriceCalculator cyclePriceCalculatorSpy = Mockito.spy(cyclePriceCalculator1);
Mockito.when(cyclePriceCalculatorSpy.findRate(anyMap(), anyString(), anyString(), anyMap(), anyInt()))
.thenReturn(subComponentMap);
String actual = cyclePriceCalculatorSpy.frameRateCalculator("diamond, steel", "2020", "jul", cycle);
assertEquals(expected, actual);
}
/**
* testFrameRateCalculator2 is to test frame type stepthrough in frameRateCalculator
*/
@SuppressWarnings("unchecked")
@Test
public void testFrameRateCalculator2() {
String expected = "500";
Frame frame = new Frame();
StepThrough[] stepThrough = new StepThrough[1];
stepThrough[0] = new StepThrough();
stepThrough[0].setYear(2020);
stepThrough[0].setCostMap(setCostMap());
frame.setStepThrough(stepThrough);
Steel[] steel = new Steel[1];
steel[0] = new Steel();
steel[0].setYear(2020);
steel[0].setCostMap(setCostMap());
frame.setSteel(steel);
cycle.setFrame(frame);
Map<String, Integer> subComponentMap = new HashMap<>();
subComponentMap.put("yearFlag", 1);
subComponentMap.put("rate", 500);
subComponentMap.put("currentIndex", 0);
subComponentMap.put("year", 2020);
CyclePriceCalculator cyclePriceCalculator1 = new CyclePriceCalculator(cycle, blockingQueue);
CyclePriceCalculator cyclePriceCalculatorSpy = Mockito.spy(cyclePriceCalculator1);
Mockito.when(cyclePriceCalculatorSpy.findRate(anyMap(), anyString(), anyString(), anyMap(), anyInt()))
.thenReturn(subComponentMap);
String actual = cyclePriceCalculatorSpy.frameRateCalculator("stepthrough, steel", "2020", "jul", cycle);
assertEquals(expected, actual);
}
/**
* testFrameRateCalculator3 is to test frame type contilever in frameRateCalculator
*/
@SuppressWarnings("unchecked")
@Test
public void testFrameRateCalculator3() {
String expected = "330";
Frame frame = new Frame();
Contilever[] contilever = new Contilever[1];
contilever[0] = new Contilever();
contilever[0].setYear(2020);
contilever[0].setCostMap(setCostMap());
frame.setContilever(contilever);
Steel[] steel = new Steel[1];
steel[0] = new Steel();
steel[0].setYear(2020);
steel[0].setCostMap(setCostMap());
frame.setSteel(steel);
cycle.setFrame(frame);
Map<String, Integer> subComponentMap = new HashMap<>();
subComponentMap.put("yearFlag", 1);
subComponentMap.put("rate", 330);
subComponentMap.put("currentIndex", 0);
subComponentMap.put("year", 2020);
CyclePriceCalculator cyclePriceCalculator1 = new CyclePriceCalculator(cycle, blockingQueue);
CyclePriceCalculator cyclePriceCalculatorSpy = Mockito.spy(cyclePriceCalculator1);
Mockito.when(cyclePriceCalculatorSpy.findRate(anyMap(), anyString(), anyString(), anyMap(), anyInt()))
.thenReturn(subComponentMap);
String actual = cyclePriceCalculatorSpy.frameRateCalculator("contilever, steel", "2020", "jul", cycle);
assertEquals(expected, actual);
}
/**
* testFrameRateCalculator4 is to test frame type recumbent in frameRateCalculator
*/
@SuppressWarnings("unchecked")
@Test
public void testFrameRateCalculator4() {
String expected = "300";
Frame frame = new Frame();
Recumbent[] recumbent = new Recumbent[1];
recumbent[0] = new Recumbent();
recumbent[0].setYear(2020);
recumbent[0].setCostMap(setCostMap());
frame.setRecumbent(recumbent);
Steel[] steel = new Steel[1];
steel[0] = new Steel();
steel[0].setYear(2020);
steel[0].setCostMap(setCostMap());
frame.setSteel(steel);
cycle.setFrame(frame);
Map<String, Integer> subComponentMap = new HashMap<>();
subComponentMap.put("yearFlag", 1);
subComponentMap.put("rate", 300);
subComponentMap.put("currentIndex", 0);
subComponentMap.put("year", 2020);
CyclePriceCalculator cyclePriceCalculator1 = new CyclePriceCalculator(cycle, blockingQueue);
CyclePriceCalculator cyclePriceCalculatorSpy = Mockito.spy(cyclePriceCalculator1);
Mockito.when(cyclePriceCalculatorSpy.findRate(anyMap(), anyString(), anyString(), anyMap(), anyInt()))
.thenReturn(subComponentMap);
String actual = cyclePriceCalculatorSpy.frameRateCalculator("recumbent, steel", "2020", "jul", cycle);
assertEquals(expected, actual);
}
/**
* testFrameRateCalculator5 is to test frame type titanium in frameRateCalculator
*/
@SuppressWarnings("unchecked")
@Test
public void testFrameRateCalculator5() {
String expected = "900";
Frame frame = new Frame();
Contilever[] contilever = new Contilever[1];
contilever[0] = new Contilever();
contilever[0].setYear(2020);
contilever[0].setCostMap(setCostMap());
frame.setContilever(contilever);
Titanium[] titanium = new Titanium[1];
titanium[0] = new Titanium();
titanium[0].setYear(2020);
titanium[0].setCostMap(setCostMap());
frame.setTitanium(titanium);
cycle.setFrame(frame);
Map<String, Integer> subComponentMap = new HashMap<>();
subComponentMap.put("yearFlag", 1);
subComponentMap.put("rate", 900);
subComponentMap.put("currentIndex", 0);
subComponentMap.put("year", 2020);
CyclePriceCalculator cyclePriceCalculator1 = new CyclePriceCalculator(cycle, blockingQueue);
CyclePriceCalculator cyclePriceCalculatorSpy = Mockito.spy(cyclePriceCalculator1);
Mockito.when(cyclePriceCalculatorSpy.findRate(anyMap(), anyString(), anyString(), anyMap(), anyInt()))
.thenReturn(subComponentMap);
String actual = cyclePriceCalculatorSpy.frameRateCalculator("titanium, contilever", "2020", "jul", cycle);
assertEquals(expected, actual);
}
/**
* testFrameRateCalculator6 is to test frame type aluminum in frameRateCalculator
*/
@SuppressWarnings("unchecked")
@Test
public void testFrameRateCalculator6() {
String expected = "900";
Frame frame = new Frame();
Aluminium[] aluminium = new Aluminium[1];
aluminium[0] = new Aluminium();
aluminium[0].setYear(2020);
aluminium[0].setCostMap(setCostMap());
frame.setAluminium(aluminium);
Diamond[] diamond = new Diamond[1];
diamond[0] = new Diamond();
diamond[0].setYear(2020);
diamond[0].setCostMap(setCostMap());
frame.setDiamond(diamond);
cycle.setFrame(frame);
Map<String, Integer> subComponentMap = new HashMap<>();
subComponentMap.put("yearFlag", 1);
subComponentMap.put("rate", 900);
subComponentMap.put("currentIndex", 0);
subComponentMap.put("year", 2020);
CyclePriceCalculator cyclePriceCalculator1 = new CyclePriceCalculator(cycle, blockingQueue);
CyclePriceCalculator cyclePriceCalculatorSpy = Mockito.spy(cyclePriceCalculator1);
Mockito.when(cyclePriceCalculatorSpy.findRate(anyMap(), anyString(), anyString(), anyMap(), anyInt()))
.thenReturn(subComponentMap);
String actual = cyclePriceCalculatorSpy.frameRateCalculator("aluminium, diamond", "2020", "jul", cycle);
assertEquals(expected, actual);
}
/**
* testFrameRateCalculator7 is test frame type steel in frameRateCalculator
*/
@SuppressWarnings("unchecked")
@Test
public void testFrameRateCalculator7() {
String expected = "550";
Frame frame = new Frame();
Recumbent[] recumbent = new Recumbent[1];
recumbent[0] = new Recumbent();
recumbent[0].setYear(2020);
recumbent[0].setCostMap(setCostMap());
frame.setRecumbent(recumbent);
Steel[] steel = new Steel[1];
steel[0] = new Steel();
steel[0].setYear(2020);
steel[0].setCostMap(setCostMap());
frame.setSteel(steel);
cycle.setFrame(frame);
Map<String, Integer> subComponentMap = new HashMap<>();
subComponentMap.put("yearFlag", 1);
subComponentMap.put("rate", 550);
subComponentMap.put("currentIndex", 0);
subComponentMap.put("year", 2020);
CyclePriceCalculator cyclePriceCalculator1 = new CyclePriceCalculator(cycle, blockingQueue);
CyclePriceCalculator cyclePriceCalculatorSpy = Mockito.spy(cyclePriceCalculator1);
Mockito.when(cyclePriceCalculatorSpy.findRate(anyMap(), anyString(), anyString(), anyMap(), anyInt()))
.thenReturn(subComponentMap);
String actual = cyclePriceCalculatorSpy.frameRateCalculator("steel, recumbent", "2020", "jul", cycle);
assertEquals(expected, actual);
}
/**
* testWheelsRateCalculator1 is test tubesless type of wheels in wheelsRateCalculator
*/
@Test
public void testWheelsRateCalculator1() {
String expected = "1650";
Wheels wheels = new Wheels();
Rim[] rim = new Rim[1];
rim[0] = new Rim();
rim[0].setYear(2020);
rim[0].setCostMap(setCostMap());
wheels.setRim(rim);
Spokes[] spokes = new Spokes[1];
spokes[0] = new Spokes();
spokes[0].setYear(2020);
spokes[0].setCostMap(setCostMap());
wheels.setSpokes(spokes);
Tube[] tube = new Tube[1];
tube[0] = new Tube();
tube[0].setYear(2020);
tube[0].setCostMap(setCostMap());
wheels.setTube(tube);
Tyre[] tyre = new Tyre[1];
tyre[0] = new Tyre();
tyre[0].setYear(2020);
tyre[0].setCostMap(setCostMap());
wheels.setTyre(tyre);
cycle.setWheels(wheels);
Map<String, Integer> subComponentMap = new HashMap<>();
subComponentMap.put("yearFlag", 1);
subComponentMap.put("rate", 550);
subComponentMap.put("currentIndex", 0);
subComponentMap.put("year", 2020);
CyclePriceCalculator cyclePriceCalculator1 = new CyclePriceCalculator(cycle, blockingQueue);
CyclePriceCalculator cyclePriceCalculatorSpy = Mockito.spy(cyclePriceCalculator1);
Mockito.when(cyclePriceCalculatorSpy.findRate(anyMap(), anyString(), anyString(), anyMap(), anyInt()))
.thenReturn(subComponentMap);
String actual = cyclePriceCalculatorSpy.wheelsRateCalculator("tubeless", "2020", "jul", cycle);
assertEquals(expected, actual);
}
/**
* testWheelsRateCalculator2 is test rimless type of wheels in wheelsRateCalculator
*/
@Test
public void testWheelsRateCalculator2() {
String expected = "1500";
Wheels wheels = new Wheels();
Rim[] rim = new Rim[1];
rim[0] = new Rim();
rim[0].setYear(2020);
rim[0].setCostMap(setCostMap());
wheels.setRim(rim);
Spokes[] spokes = new Spokes[1];
spokes[0] = new Spokes();
spokes[0].setYear(2020);
spokes[0].setCostMap(setCostMap());
wheels.setSpokes(spokes);
Tube[] tube = new Tube[1];
tube[0] = new Tube();
tube[0].setYear(2020);
tube[0].setCostMap(setCostMap());
wheels.setTube(tube);
Tyre[] tyre = new Tyre[1];
tyre[0] = new Tyre();
tyre[0].setYear(2020);
tyre[0].setCostMap(setCostMap());
wheels.setTyre(tyre);
cycle.setWheels(wheels);
Map<String, Integer> subComponentMap = new HashMap<>();
subComponentMap.put("yearFlag", 1);
subComponentMap.put("rate", 500);
subComponentMap.put("currentIndex", 0);
subComponentMap.put("year", 2020);
CyclePriceCalculator cyclePriceCalculator1 = new CyclePriceCalculator(cycle, blockingQueue);
CyclePriceCalculator cyclePriceCalculatorSpy = Mockito.spy(cyclePriceCalculator1);
Mockito.when(cyclePriceCalculatorSpy.findRate(anyMap(), anyString(), anyString(), anyMap(), anyInt()))
.thenReturn(subComponentMap);
String actual = cyclePriceCalculatorSpy.wheelsRateCalculator("rimless", "2020", "jul", cycle);
assertEquals(expected, actual);
}
/**
* testWheelsRateCalculator3 is test spokeless type of wheels in wheelsRateCalculator
*/
@Test
public void testWheelsRateCalculator3() {
String expected = "1200";
Wheels wheels = new Wheels();
Rim[] rim = new Rim[1];
rim[0] = new Rim();
rim[0].setYear(2020);
rim[0].setCostMap(setCostMap());
wheels.setRim(rim);
Spokes[] spokes = new Spokes[1];
spokes[0] = new Spokes();
spokes[0].setYear(2020);
spokes[0].setCostMap(setCostMap());
wheels.setSpokes(spokes);
Tube[] tube = new Tube[1];
tube[0] = new Tube();
tube[0].setYear(2020);
tube[0].setCostMap(setCostMap());
wheels.setTube(tube);
Tyre[] tyre = new Tyre[1];
tyre[0] = new Tyre();
tyre[0].setYear(2020);
tyre[0].setCostMap(setCostMap());
wheels.setTyre(tyre);
cycle.setWheels(wheels);
Map<String, Integer> subComponentMap = new HashMap<>();
subComponentMap.put("yearFlag", 1);
subComponentMap.put("rate", 400);
subComponentMap.put("currentIndex", 0);
subComponentMap.put("year", 2020);
CyclePriceCalculator cyclePriceCalculator1 = new CyclePriceCalculator(cycle, blockingQueue);
CyclePriceCalculator cyclePriceCalculatorSpy = Mockito.spy(cyclePriceCalculator1);
Mockito.when(cyclePriceCalculatorSpy.findRate(anyMap(), anyString(), anyString(), anyMap(), anyInt()))
.thenReturn(subComponentMap);
String actual = cyclePriceCalculatorSpy.wheelsRateCalculator("spokeless", "2020", "jul", cycle);
assertEquals(expected, actual);
}
/**
* testChainAssemblyRateCalculator1 is to test fixed gear in chainAssemblyRateCalculator
*/
@Test
public void testChainAssemblyRateCalculator1() {
String expected = "520";
ChainAssembly chainAssembly = new ChainAssembly();
FixedGear[] fixed = new FixedGear[1];
fixed[0] = new FixedGear();
fixed[0].setYear(2020);
fixed[0].setCostMap(setCostMap());
chainAssembly.setFixedGear(fixed);
cycle.setChainAssembly(chainAssembly);
Map<String, Integer> subComponentMap = new HashMap<>();
subComponentMap.put("yearFlag", 1);
subComponentMap.put("rate", 520);
subComponentMap.put("currentIndex", 0);
subComponentMap.put("year", 2020);
CyclePriceCalculator cyclePriceCalculator1 = new CyclePriceCalculator(cycle, blockingQueue);
CyclePriceCalculator cyclePriceCalculatorSpy = Mockito.spy(cyclePriceCalculator1);
Mockito.when(cyclePriceCalculatorSpy.findRate(anyMap(), anyString(), anyString(), anyMap(), anyInt()))
.thenReturn(subComponentMap);
String actual = cyclePriceCalculatorSpy.chainAssemblyRateCalculator("fixed", "2020", "jul", cycle);
assertEquals(expected, actual);
}
/**
* testChainAssemblyRateCalculator2 is to test gear3 in chainAssemblyRateCalculator
*/
@Test
public void testChainAssemblyRateCalculator2() {
String expected = "600";
ChainAssembly chainAssembly = new ChainAssembly();
Gear3[] gear3 = new Gear3[1];
gear3[0] = new Gear3();
gear3[0].setYear(2020);
gear3[0].setCostMap(setCostMap());
chainAssembly.setGear3(gear3);
cycle.setChainAssembly(chainAssembly);
Map<String, Integer> subComponentMap = new HashMap<>();
subComponentMap.put("yearFlag", 1);
subComponentMap.put("rate", 600);
subComponentMap.put("currentIndex", 0);
subComponentMap.put("year", 2020);
CyclePriceCalculator cyclePriceCalculator1 = new CyclePriceCalculator(cycle, blockingQueue);
CyclePriceCalculator cyclePriceCalculatorSpy = Mockito.spy(cyclePriceCalculator1);
Mockito.when(cyclePriceCalculatorSpy.findRate(anyMap(), anyString(), anyString(), anyMap(), anyInt()))
.thenReturn(subComponentMap);
String actual = cyclePriceCalculatorSpy.chainAssemblyRateCalculator("gear3", "2020", "jul", cycle);
assertEquals(expected, actual);
}
/**
* testChainAssemblyRateCalculator3 is to test gear4 in chainAssemblyRateCalculator
*/
@Test
public void testChainAssemblyRateCalculator3() {
String expected = "650";
ChainAssembly chainAssembly = new ChainAssembly();
Gear4[] gear4 = new Gear4[1];
gear4[0] = new Gear4();
gear4[0].setYear(2020);
gear4[0].setCostMap(setCostMap());
chainAssembly.setGear4(gear4);
cycle.setChainAssembly(chainAssembly);
Map<String, Integer> subComponentMap = new HashMap<>();
subComponentMap.put("yearFlag", 1);
subComponentMap.put("rate", 650);
subComponentMap.put("currentIndex", 0);
subComponentMap.put("year", 2020);
CyclePriceCalculator cyclePriceCalculator1 = new CyclePriceCalculator(cycle, blockingQueue);
CyclePriceCalculator cyclePriceCalculatorSpy = Mockito.spy(cyclePriceCalculator1);
Mockito.when(cyclePriceCalculatorSpy.findRate(anyMap(), anyString(), anyString(), anyMap(), anyInt()))
.thenReturn(subComponentMap);
String actual = cyclePriceCalculatorSpy.chainAssemblyRateCalculator("gear4", "2020", "jul", cycle);
assertEquals(expected, actual);
}
/**
* testChainAssemblyRateCalculator4 is to test gear5 in chainAssemblyRateCalculator
*/
@Test
public void testChainAssemblyRateCalculator4() {
String expected = "670";
ChainAssembly chainAssembly = new ChainAssembly();
Gear5[] gear5 = new Gear5[1];
gear5[0] = new Gear5();
gear5[0].setYear(2020);
gear5[0].setCostMap(setCostMap());
chainAssembly.setGear5(gear5);
cycle.setChainAssembly(chainAssembly);
Map<String, Integer> subComponentMap = new HashMap<>();
subComponentMap.put("yearFlag", 1);
subComponentMap.put("rate", 670);
subComponentMap.put("currentIndex", 0);
subComponentMap.put("year", 2020);
CyclePriceCalculator cyclePriceCalculator1 = new CyclePriceCalculator(cycle, blockingQueue);
CyclePriceCalculator cyclePriceCalculatorSpy = Mockito.spy(cyclePriceCalculator1);
Mockito.when(cyclePriceCalculatorSpy.findRate(anyMap(), anyString(), anyString(), anyMap(), anyInt()))
.thenReturn(subComponentMap);
String actual = cyclePriceCalculatorSpy.chainAssemblyRateCalculator("gear5", "2020", "jul", cycle);
assertEquals(expected, actual);
}
/**
* testChainAssemblyRateCalculator5 is to test gear6 in chainAssemblyRateCalculator
*/
@Test
public void testChainAssemblyRateCalculator5() {
String expected = "680";
ChainAssembly chainAssembly = new ChainAssembly();
Gear6[] gear6 = new Gear6[1];
gear6[0] = new Gear6();
gear6[0].setYear(2020);
gear6[0].setCostMap(setCostMap());
chainAssembly.setGear6(gear6);
cycle.setChainAssembly(chainAssembly);
Map<String, Integer> subComponentMap = new HashMap<>();
subComponentMap.put("yearFlag", 1);
subComponentMap.put("rate", 680);
subComponentMap.put("currentIndex", 0);
subComponentMap.put("year", 2020);
CyclePriceCalculator cyclePriceCalculator1 = new CyclePriceCalculator(cycle, blockingQueue);
CyclePriceCalculator cyclePriceCalculatorSpy = Mockito.spy(cyclePriceCalculator1);
Mockito.when(cyclePriceCalculatorSpy.findRate(anyMap(), anyString(), anyString(), anyMap(), anyInt()))
.thenReturn(subComponentMap);
String actual = cyclePriceCalculatorSpy.chainAssemblyRateCalculator("gear6", "2020", "jul", cycle);
assertEquals(expected, actual);
}
/**
* testChainAssemblyRateCalculator6 is to test gear7 in chainAssemblyRateCalculator
*/
@Test
public void testChainAssemblyRateCalculator6() {
String expected = "680";
ChainAssembly chainAssembly = new ChainAssembly();
Gear7[] gear7 = new Gear7[1];
gear7[0] = new Gear7();
gear7[0].setYear(2020);
gear7[0].setCostMap(setCostMap());
chainAssembly.setGear7(gear7);
cycle.setChainAssembly(chainAssembly);
Map<String, Integer> subComponentMap = new HashMap<>();
subComponentMap.put("yearFlag", 1);
subComponentMap.put("rate", 680);
subComponentMap.put("currentIndex", 0);
subComponentMap.put("year", 2020);
CyclePriceCalculator cyclePriceCalculator1 = new CyclePriceCalculator(cycle, blockingQueue);
CyclePriceCalculator cyclePriceCalculatorSpy = Mockito.spy(cyclePriceCalculator1);
Mockito.when(cyclePriceCalculatorSpy.findRate(anyMap(), anyString(), anyString(), anyMap(), anyInt()))
.thenReturn(subComponentMap);
String actual = cyclePriceCalculatorSpy.chainAssemblyRateCalculator("gear7", "2020", "jul", cycle);
assertEquals(expected, actual);
}
/**
* testChainAssemblyRateCalculator7 is to test gear8 in chainAssemblyRateCalculator
*/
@Test
public void testChainAssemblyRateCalculator7() {
String expected = "680";
ChainAssembly chainAssembly = new ChainAssembly();
Gear8[] gear8 = new Gear8[1];
gear8[0] = new Gear8();
gear8[0].setYear(2020);
gear8[0].setCostMap(setCostMap());
chainAssembly.setGear8(gear8);
cycle.setChainAssembly(chainAssembly);
Map<String, Integer> subComponentMap = new HashMap<>();
subComponentMap.put("yearFlag", 1);
subComponentMap.put("rate", 680);
subComponentMap.put("currentIndex", 0);
subComponentMap.put("year", 2020);
CyclePriceCalculator cyclePriceCalculator1 = new CyclePriceCalculator(cycle, blockingQueue);
CyclePriceCalculator cyclePriceCalculatorSpy = Mockito.spy(cyclePriceCalculator1);
Mockito.when(cyclePriceCalculatorSpy.findRate(anyMap(), anyString(), anyString(), anyMap(), anyInt()))
.thenReturn(subComponentMap);
String actual = cyclePriceCalculatorSpy.chainAssemblyRateCalculator("gear8", "2020", "jul", cycle);
assertEquals(expected, actual);
}
/**
* testSeatingRateCalculator1 is to test cruiser saddle in seatingRateCalculator
*/
@Test
public void testSeatingRateCalculator1() {
String expected = "560";
Seating seating = new Seating();
CruiserSaddle[] cruiser = new CruiserSaddle[1];
cruiser[0] = new CruiserSaddle();
cruiser[0].setYear(2020);
cruiser[0].setCostMap(setCostMap());
seating.setCruiserSaddle(cruiser);
cycle.setSeating(seating);
Map<String, Integer> subComponentMap = new HashMap<>();
subComponentMap.put("yearFlag", 1);
subComponentMap.put("rate", 560);
subComponentMap.put("currentIndex", 0);
subComponentMap.put("year", 2020);
CyclePriceCalculator cyclePriceCalculator1 = new CyclePriceCalculator(cycle, blockingQueue);
CyclePriceCalculator cyclePriceCalculatorSpy = Mockito.spy(cyclePriceCalculator1);
Mockito.when(cyclePriceCalculatorSpy.findRate(anyMap(), anyString(), anyString(), anyMap(), anyInt()))
.thenReturn(subComponentMap);
String actual = cyclePriceCalculatorSpy.seatingRateCalculator("cruiser", "2020", "jul", cycle);
assertEquals(expected, actual);
}
/**
* testSeatingRateCalculator2 is to test racing saddle in seatingRateCalculator
*/
@Test
public void testSeatingRateCalculator2() {
String expected = "590";
Seating seating = new Seating();
RacingSaddle[] racing = new RacingSaddle[1];
racing[0] = new RacingSaddle();
racing[0].setYear(2020);
racing[0].setCostMap(setCostMap());
seating.setRacingSaddle(racing);
cycle.setSeating(seating);
Map<String, Integer> subComponentMap = new HashMap<>();
subComponentMap.put("yearFlag", 1);
subComponentMap.put("rate", 590);
subComponentMap.put("currentIndex", 0);
subComponentMap.put("year", 2020);
CyclePriceCalculator cyclePriceCalculator1 = new CyclePriceCalculator(cycle, blockingQueue);
CyclePriceCalculator cyclePriceCalculatorSpy = Mockito.spy(cyclePriceCalculator1);
Mockito.when(cyclePriceCalculatorSpy.findRate(anyMap(), anyString(), anyString(), anyMap(), anyInt()))
.thenReturn(subComponentMap);
String actual = cyclePriceCalculatorSpy.seatingRateCalculator("racing", "2020", "jul", cycle);
assertEquals(expected, actual);
}
/**
* testSeatingRateCalculator3 is to test comfort saddle in seatingRateCalculator
*/
@Test
public void testSeatingRateCalculator3() {
String expected = "650";
Seating seating = new Seating();
ComfortSaddle[] comfort = new ComfortSaddle[1];
comfort[0] = new ComfortSaddle();
comfort[0].setYear(2020);
comfort[0].setCostMap(setCostMap());
seating.setComfortSaddle(comfort);
cycle.setSeating(seating);
Map<String, Integer> subComponentMap = new HashMap<>();
subComponentMap.put("yearFlag", 1);
subComponentMap.put("rate", 650);
subComponentMap.put("currentIndex", 0);
subComponentMap.put("year", 2020);
CyclePriceCalculator cyclePriceCalculator1 = new CyclePriceCalculator(cycle, blockingQueue);
CyclePriceCalculator cyclePriceCalculatorSpy = Mockito.spy(cyclePriceCalculator1);
Mockito.when(cyclePriceCalculatorSpy.findRate(anyMap(), anyString(), anyString(), anyMap(), anyInt()))
.thenReturn(subComponentMap);
String actual = cyclePriceCalculatorSpy.seatingRateCalculator("comfort", "2020", "jul", cycle);
assertEquals(expected, actual);
}
/**
* testHandlBarRateCalculator1 is to test drop handle bar in handlBarRateCalculator
*/
@Test
public void testHandlBarRateCalculator1() {
String expected = "700";
HandleBar handleBar = new HandleBar();
DropBar[] dropBar = new DropBar[1];
dropBar[0] = new DropBar();
dropBar[0].setYear(2020);
dropBar[0].setCostMap(setCostMap());
handleBar.setDropBar(dropBar);
cycle.setHandleBar(handleBar);
Map<String, Integer> subComponentMap = new HashMap<>();
subComponentMap.put("yearFlag", 1);
subComponentMap.put("rate", 700);
subComponentMap.put("currentIndex", 0);
subComponentMap.put("year", 2020);
CyclePriceCalculator cyclePriceCalculator1 = new CyclePriceCalculator(cycle, blockingQueue);
CyclePriceCalculator cyclePriceCalculatorSpy = Mockito.spy(cyclePriceCalculator1);
Mockito.when(cyclePriceCalculatorSpy.findRate(anyMap(), anyString(), anyString(), anyMap(), anyInt()))
.thenReturn(subComponentMap);
String actual = cyclePriceCalculatorSpy.handleBarRateCalculator("drop", "2020", "jul", cycle);
assertEquals(expected, actual);
}
/**
* testHandlBarRateCalculator2 is to test pursuit handle bar in handlBarRateCalculator
*/
@Test
public void testHandlBarRateCalculator2() {
String expected = "700";
HandleBar handleBar = new HandleBar();
PursuitBar[] pursuitBar = new PursuitBar[1];
pursuitBar[0] = new PursuitBar();
pursuitBar[0].setYear(2020);
pursuitBar[0].setCostMap(setCostMap());
handleBar.setPursuitBar(pursuitBar);
cycle.setHandleBar(handleBar);
Map<String, Integer> subComponentMap = new HashMap<>();
subComponentMap.put("yearFlag", 1);
subComponentMap.put("rate", 700);
subComponentMap.put("currentIndex", 0);
subComponentMap.put("year", 2020);
CyclePriceCalculator cyclePriceCalculator1 = new CyclePriceCalculator(cycle, blockingQueue);
CyclePriceCalculator cyclePriceCalculatorSpy = Mockito.spy(cyclePriceCalculator1);
Mockito.when(cyclePriceCalculatorSpy.findRate(anyMap(), anyString(), anyString(), anyMap(), anyInt()))
.thenReturn(subComponentMap);
String actual = cyclePriceCalculatorSpy.handleBarRateCalculator("pursuit", "2020", "jul", cycle);
assertEquals(expected, actual);
}
/**
* testHandlBarRateCalculator3 is to test riser handle bar in handlBarRateCalculator
*/
@Test
public void testHandlBarRateCalculator3() {
String expected = "700";
HandleBar handleBar = new HandleBar();
RiserBar[] riserBar = new RiserBar[1];
riserBar[0] = new RiserBar();
riserBar[0].setYear(2020);
riserBar[0].setCostMap(setCostMap());
handleBar.setRiserBar(riserBar);
cycle.setHandleBar(handleBar);
Map<String, Integer> subComponentMap = new HashMap<>();
subComponentMap.put("yearFlag", 1);
subComponentMap.put("rate", 700);
subComponentMap.put("currentIndex", 0);
subComponentMap.put("year", 2020);
CyclePriceCalculator cyclePriceCalculator1 = new CyclePriceCalculator(cycle, blockingQueue);
CyclePriceCalculator cyclePriceCalculatorSpy = Mockito.spy(cyclePriceCalculator1);
Mockito.when(cyclePriceCalculatorSpy.findRate(anyMap(), anyString(), anyString(), anyMap(), anyInt()))
.thenReturn(subComponentMap);
String actual = cyclePriceCalculatorSpy.handleBarRateCalculator("riser", "2020", "jul", cycle);
assertEquals(expected, actual);
}
}<file_sep>/Family Tree/README.txt
Family tree program is to model out the King Shan family so that :
- Given a 'name' and a 'relationship', the people corresponding to that relationship in the order in which they were added to the family will be displayed asssuming the names of the family members are unique.
- Program will enable us to add a child to any family in the tree through the mother.
System Requirements:
---------------------
- System must have java-8 or higher version.
- System must comply minimum requirements specified for jvm.
Libraries added in the application
-----------------------------------
- junit-4.13.jar
- Used for unit testing of the code. All Unit tests are written in TestGeekTrust.java, which can be run using TestRunner.java.
- hamcrest-core-1.3.jar
- Compile time dependency of Junit.
- mockito-all-1.9.5.jar
- Used to create and configure mock objects which simplifies the development of tests for classes with external dependencies.
Running in an IDE
---------------------
If you want to run the application in an IDE, such as Eclipse, copy the code and add the corresponding dependencies into classpath and then run FamilyTree.java to run application or TestFamilyTree.java to run the tests.
Execution instructions:
--------------------------
- Program will take the location to the test file as the input paramter.
- Input will be read from a text file.
- Corresponding output to the test file will be displayed in the console.
Relationships handled:
-----------------------
Paternal-Uncle
Maternal-Uncle
Paternal-Aunt
Maternal-Aunt
Sister-In-Law
Brother-In-Law
Son
Daughter
Siblings
Inputs to the application:
-------------------------
- To add child
ADD_CHILD Tritha Aarohi Female
- To search a relation
GET_RELATIONSHIP Vasa Siblings
Assumptions:
-------------
- Existing family tree in the problem statement will be initialised during the start of the program.
- Each person who is added in family tree must have parent associated with it except head of the family.
- All Persons should have a unique name in the tree.
- A member can only be added with an existing member only.
<file_sep>/Family Tree/TestFamilyTree.java
package family;
import static org.junit.Assert.assertEquals;
import static org.mockito.Mockito.times;
import java.util.ArrayList;
import java.util.List;
import org.junit.Test;
import org.mockito.Mockito;
/**
* Testcases to test Geektrust.java
* @author Akshara
*/
public class TestFamilyTree {
RelationHandler relationHandler = new RelationHandler();
RelationHandler relationHandlerMock = Mockito.mock(RelationHandler.class);
Person head = new Person("Shan", Gender.MALE);
/**
* Tests method buildShanFamily()
*/
@Test
public void testBuildShanFamily() {
Person expectedPerson = new Person("Shan", Gender.MALE);
Person actualPerson = relationHandler.buildShanFamily();
assertEquals(expectedPerson.getName(), actualPerson.getName());
assertEquals(expectedPerson.getGender(), actualPerson.getGender());
}
/**
* tests method searchMember()
*/
@Test
public void testSearchMember() {
Person expectedPerson = new Person("Shan", Gender.MALE);
Person actualPerson = relationHandler.searchMember(head, "Shan");
assertEquals(expectedPerson.getName(), actualPerson.getName());
assertEquals(expectedPerson.getGender(), actualPerson.getGender());
assertEquals(expectedPerson.getFamily(), actualPerson.getFamily());
assertEquals(expectedPerson.getParentFamily(), actualPerson.getParentFamily());
}
/**
* tests method addMember()
*/
@Test
public void testAddMember() {
Mockito.doNothing().when(relationHandlerMock)
.addMember("Anga", "Shah", "Male", head);
relationHandlerMock.addMember("Anga", "Shah", "Male", head);
Mockito.verify(relationHandlerMock, times(1)).addMember("Anga", "Shah", "Male", head);
}
/**
* tests method printList()
*/
@Test
public void testPrintList() {
Mockito.doNothing().when(relationHandlerMock).printList(new ArrayList<>());
relationHandlerMock.printList(new ArrayList<>());
Mockito.verify(relationHandlerMock, times(1)).printList(new ArrayList<>());
}
/**
* Tests method getKids() for son
*/
@Test
public void testGetKidsSon() {
List<Person> sonsList = new ArrayList<>();
head.addMarriage(new Person("Anga", Gender.FEMALE));
head.addSon("Chit");
head.addDaughter("Satya");
sonsList.add(relationHandler.searchMember(head, "Chit"));
List<Person> actualSonList = relationHandler.getKids(head, Gender.MALE);
assertEquals(sonsList, actualSonList);
}
/**
* Tests method getKids() for daughter
*/
@Test
public void testGetKidsDaughter() {
List<Person> daughtersList = new ArrayList<>();
head.addMarriage(new Person("Anga", Gender.FEMALE));
head.addSon("Chit");
head.addDaughter("Satya");
daughtersList.add(relationHandler.searchMember(head, "Satya"));
List<Person> actualDaughtersList = relationHandler.getKids(head, Gender.FEMALE);
assertEquals(daughtersList, actualDaughtersList);
}
/**
* Tests the result if there is no children
*/
@Test
public void testGetKidsNone() {
List<Person> kidsList = new ArrayList<>();
head.addMarriage(new Person("Anga", Gender.FEMALE));
List<Person> actualDaughtersList = relationHandler.getKids(head, Gender.FEMALE);
assertEquals(kidsList, actualDaughtersList);
}
/**
* Tests method getAuntUncle for Paternal-uncle
*/
@Test
public void testGetUncle() {
List<Person> uncleList = new ArrayList<>();
head.addMarriage(new Person("Anga", Gender.FEMALE));
head.addSon("Chit");
head.addDaughter("Satya");
Person satya = relationHandler.searchMember(head, "Satya");
satya.addMarriage(new Person("Vyan", Gender.MALE));
satya.addSon("Asva");
uncleList.add(relationHandler.searchMember(head, "Chit"));
List<Person> actualUncleList = relationHandler.getAuntUncle(satya, Gender.MALE);
assertEquals(uncleList, actualUncleList);
}
/**
* Tests method getAuntUncle for Maternal-aunt
*/
@Test
public void testGetAunt() {
List<Person> auntList = new ArrayList<>();
head.addMarriage(new Person("Anga", Gender.FEMALE));
head.addSon("Chit");
head.addDaughter("Satya");
Person chit = relationHandler.searchMember(head, "Chit");
chit.addMarriage(new Person("Amba", Gender.FEMALE));
chit.addDaughter("Dritha");
auntList.add(relationHandler.searchMember(head, "Satya"));
List<Person> actualAuntList = relationHandler.getAuntUncle(chit, Gender.FEMALE);
assertEquals(auntList, actualAuntList);
}
/**
* Tests method testGetBrotherInLaw() for bother-in-law
*/
@Test
public void testGetBrotherInLaw() {
List<Person> brotherInLawList = new ArrayList<>();
head.addMarriage(new Person("Anga", Gender.FEMALE));
head.addSon("Chit");
head.addDaughter("Satya");
Person satya = relationHandler.searchMember(head, "Satya");
satya.addMarriage(new Person("Vyan", Gender.MALE));
brotherInLawList.add(relationHandler.searchMember(head, "Chit"));
Person vyan = relationHandler.searchMember(head, "Vyan");
List<Person> actualBrotherInLawList = relationHandler.getInLaws(vyan, Gender.MALE);
assertEquals(brotherInLawList, actualBrotherInLawList);
}
/**
* Tests method testGetBrotherInLaw() for sister-in-law
*/
@Test
public void testGetSisterInLaw() {
List<Person> sisterInLawList = new ArrayList<>();
head.addMarriage(new Person("Anga", Gender.FEMALE));
head.addSon("Chit");
head.addDaughter("Satya");
Person chit = relationHandler.searchMember(head, "Chit");
chit.addMarriage(new Person("Amba", Gender.FEMALE));
sisterInLawList.add(relationHandler.searchMember(head, "Satya"));
Person amba = relationHandler.searchMember(head, "Amba");
List<Person> actualSisterInLawList = relationHandler.getInLaws(amba, Gender.FEMALE);
assertEquals(sisterInLawList, actualSisterInLawList);
}
/**
* Tests method testGetRelation()
*/
@Test
public void testGetRelation() {
Mockito.doNothing().when(relationHandlerMock).getRelation("", "", head);
relationHandlerMock.getRelation("", "", head);
Mockito.verify(relationHandlerMock, times(1)).getRelation("", "", head);
}
}
<file_sep>/CyclePriceCalculator/Model/Components/Wheels/Wheels.java
package Model.Components.Wheels;
public class Wheels {
private Spokes[] spokes;
private Rim[] rim;
private Tube[] tube;
private Tyre[] tyre;
public synchronized Spokes[] getSpokes() {
return spokes;
}
public synchronized void setSpokes(Spokes[] spokes) {
this.spokes = spokes;
}
public synchronized Rim[] getRim() {
return rim;
}
public synchronized void setRim(Rim[] rim) {
this.rim = rim;
}
public synchronized Tube[] getTube() {
return tube;
}
public synchronized void setTube(Tube[] tube) {
this.tube = tube;
}
public synchronized Tyre[] getTyre() {
return tyre;
}
public synchronized void setTyre(Tyre[] tyre) {
this.tyre = tyre;
}
}
| 8632541d3cfec3c050710e9e69a740e3888a2fb7 | [
"Java",
"Text"
] | 10 | Java | AKSHARA-T-S-B/javaProject | 188f03cdd8afb000c77b132416813dea4b610c74 | f99bea76065ef4a2bbee4fc893988109dda1619f | |
refs/heads/master | <file_sep>filteredArray = []
function buildImages() {
url = '/fetch/api'
d3.json(url).then(data => {
// d3.select(".image_container").remove()
var input = d3.select("#input").property("value");
var input2 = d3.select("#input2").property("value");
var color_input = d3.select("#color").property("value");
var inputs = [input, input2]
filteredArray = data;
// test if both inputs are empy to display blank page
if (input === '' && input2 === '') {
filteredArray = []
}
inputs.forEach( (filter, index) => {
if (filter) {
// filter based on class from user input
filteredArray = filteredArray.filter((dict) => dict['classes_detected'].includes(filter));
// filter out anything with a certainty less than 99%
filteredArray = filteredArray.filter( (dict) => {
index_of_score = dict['classes_detected'].indexOf(filter);
return dict['scores'][index_of_score] > 0.99
})
}
});
// filteredArray = filteredArray.slice(2);
// filteredArray = colorFilter(filteredArray);
createImageDivs(filteredArray);
});
}
function colorFilter() {
var color_input = d3.select("#color").property("value");
// filter for color
switch(color_input) {
case 'red':
coloredArray = filteredArray.filter((dict) => dict['color_percentages'].red > 50);
break;
case 'blue':
coloredArray = filteredArray.filter((dict) => dict['color_percentages'].blue > 50);
break;
case 'purple':
coloredArray = filteredArray.filter((dict) => dict['color_percentages'].purple > 50);
break;
case 'orange':
coloredArray = filteredArray.filter((dict) => dict['color_percentages'].orange > 50);
break;
default:
coloredArray = filteredArray;
}
createImageDivs(coloredArray)
}
function createImageDivs(filteredArray) {
d3.select(".image_container").remove()
var container = d3.select("main")
.append("div")
.attr("class", "image_container container-fluid");
container.selectAll('img')
.data(filteredArray)
.enter()
.append('div')
.attr('class','col')
.append('div')
.attr('class','thumbnail')
.append('img')
.attr('class', 'img-fluid img-thumbnail')
.attr('src', d => d.image_url);
}
function buildPieChart(img_url) {
var data = filteredArray.filter((dict) => dict.image_url === img_url);
var colors = [];
var values = [];
var color_object = data[0]['color_percentages'];
var colorData = {'colors': colors, 'values':values};
Object.entries(color_object).forEach(([key,value]) => {
colors.push(key);
values.push(value);
});
var myDuration = 600;
var firstTime = true;
var width = 960,
height = 500,
radius = Math.min(width, height) / 2;
var width = 960,
height = 500,
radius = Math.min(width, height) / 2;
var color = d3.scaleOrdinal(d3.schemeCategory10);
var pie = d3.pie()
.value(function(d) { return colorData.values; })
.sort(null);
var arc = d3.arc()
.innerRadius(radius - 100)
.outerRadius(radius - 20);
var svg = d3.select("body").append("svg")
.attr("width", width)
.attr("height", height)
.append("g")
.attr("transform", "translate(" + width / 2 + "," + height / 2 + ")");
var colorsByValue = d3.nest()
.key(function(d) { return colorData.colors; })
.entries(colorData)
.reverse();
var label = d3.select("form").selectAll("label")
.data(colorsByValue)
.enter().append("label");
label.append("input")
.attr("type", "radio")
.attr("name", "fruit")
.attr("value", function(d) { return d.key; })
.on("change", change)
.filter(function(d, i) { return !i; })
.each(change)
.property("checked", true);
label.append("span")
.text(function(d) { return d.key; });
function change(region) {
var path = svg.selectAll("path");
var data0 = path.data(),
data1 = pie(region.values);
path = path.data(data1, key);
path
.transition()
.duration(myDuration)
.attrTween("d", arcTween)
path
.enter()
.append("path")
.each(function(d, i) {
var narc = findNeighborArc(i, data0, data1, key) ;
if(narc) {
this._current = narc;
this._previous = narc;
} else {
this._current = d;
}
})
.attr("fill", function(d,i) {
return color(d.data.region)
})
.transition()
.duration(myDuration)
.attrTween("d", arcTween)
path
.exit()
.transition()
.duration(myDuration)
.attrTween("d", function(d, index) {
var currentIndex = this._previous.data.region;
var i = d3.interpolateObject(d,this._previous);
return function(t) {
return arc(i(t))
}
})
.remove()
}
function key(d) {
return d.data.region;
}
function type(d) {
d.count = +d.count;
return d;
}
function findNeighborArc(i, data0, data1, key) {
var d;
if(d = findPreceding(i, data0, data1, key)) {
var obj = cloneObj(d)
obj.startAngle = d.endAngle;
return obj;
} else if(d = findFollowing(i, data0, data1, key)) {
var obj = cloneObj(d)
obj.endAngle = d.startAngle;
return obj;
}
return null
}
// Find the element in data0 that joins the highest preceding element in data1.
function findPreceding(i, data0, data1, key) {
var m = data0.length;
while (--i >= 0) {
var k = key(data1[i]);
for (var j = 0; j < m; ++j) {
if (key(data0[j]) === k) return data0[j];
}
}
}
// Find the element in data0 that joins the lowest following element in data1.
function findFollowing(i, data0, data1, key) {
var n = data1.length, m = data0.length;
while (++i < n) {
var k = key(data1[i]);
for (var j = 0; j < m; ++j) {
if (key(data0[j]) === k) return data0[j];
}
}
}
function arcTween(d) {
var i = d3.interpolate(this._current, d);
this._current = i(0);
return function(t) {
return arc(i(t))
}
}
function cloneObj(obj) {
var o = {};
for(var i in obj) {
o[i] = obj[i];
}
return o;
}
}
// added a bunch of event listeners because I can't figure out which one works best
d3.select("#color").on("change", colorFilter);
// d3.select("body").on("click", colorFilter);
d3.select("body").on("keydown", buildImages);
d3.select("form").on("keydown", buildImages);
d3.select("html").on("keydown", buildImages);
d3.select("html").on("click", colorFilter);
// buildImages()<file_sep>from flask import Flask, render_template, request, jsonify, redirect
import pymongo
import os
from maskImage import *
from videoToImage import videoToImage
app = Flask(__name__)
stored_object = {}
output_images = []
APP_ROOT = os.path.abspath("Upload_Scrape_Mongo_Push/")
target = os.path.join(APP_ROOT, 'static','images')
output_dir = os.path.join(APP_ROOT, 'static','output')
videos_out = os.path.join(APP_ROOT, 'static','processedVideoImage')
# output_dir = 'static/output'
if not os.path.isdir(target):
os.mkdir(target)
else:
print("Couldn't create upload directory: {}".format(target))
if not os.path.isdir(output_dir):
os.mkdir(output_dir)
else:
print("Couldn't create output directory: {}".format(output_dir))
@app.route("/")
def uploadfiles():
output_images = ['static/output/' + img for img in os.listdir(output_dir)]
print(output_images)
output_videos = ['static/processedVideoImage/' + img for img in os.listdir(videos_out)]
return render_template('upload.html', output_images=output_images, output_videos=output_videos)
@app.route('/saveimages', methods=["POST"])
def saveimages():
model = startModel()
for upload in request.files.getlist("file"):
print("{} is the filename".format(upload.filename))
filename = upload.filename
destination = os.path.join(target, filename)
# destination = "/".join([target, filename])
print("Accept incoming file:", filename)
# image_path = target + upload.filename
# print("Save it to:", destination)
upload.save(destination)
file_name_root = upload.filename.split('.')[0]
# create an output file name
file_output_name = file_name_root + '_output.png'
# append file output name for later
output_images.append(file_output_name)
# run ML function
output_name = os.path.join(output_dir, file_output_name)
maskImage(destination, model, output_name)
# os.remove(destination)
return redirect("/")
@app.route('/savevideos', methods=['POST'])
def savevideos():
# redirect to home page
target = os.path.join(APP_ROOT, 'static', 'videoToImage')
for upload in request.files.getlist("file"):
print("{} is the filename".format(upload.filename))
filename = upload.filename
destination = os.path.join(target, filename)
print("Accept incoming file:", filename)
# image_path = 'static/videoToImage/' + upload.filename
# print("Save it to:", destination)
upload.save(destination)
# file_name_root = upload.filename.split('.')[0]
# create an output file name
# file_output_name = file_name_root + '_output.png'
# append file output name for later
# output_images.append(file_output_name)
# run videoToImage
videoToImage(destination)
return redirect("/")
if __name__ == "__main__":
app.run(debug=False)<file_sep>def scrape_images():
from google_images_download2.google_images_download import google_images_download
# redirect sys.stdout to a buffer
class_names = ['BG', 'person', 'bicycle', 'car', 'motorcycle', 'airplane',
'bus', 'train', 'truck', 'boat', 'traffic light',
'fire hydrant', 'stop sign', 'parking meter', 'bench', 'bird',
'cat', 'dog', 'horse', 'sheep', 'cow', 'elephant', 'bear',
'zebra', 'giraffe', 'backpack', 'umbrella', 'handbag', 'tie',
'suitcase', 'frisbee', 'skis', 'snowboard', 'sports ball',
'kite', 'baseball bat', 'baseball glove', 'skateboard',
'surfboard', 'tennis racket', 'bottle', 'wine glass', 'cup',
'fork', 'knife', 'spoon', 'bowl', 'banana', 'apple',
'sandwich', 'orange', 'broccoli', 'carrot', 'hot dog', 'pizza',
'donut', 'cake', 'chair', 'couch', 'potted plant', 'bed',
'dining table', 'toilet', 'tv', 'laptop', 'mouse', 'remote',
'keyboard', 'cell phone', 'microwave', 'oven', 'toaster',
'sink', 'refrigerator', 'book', 'clock', 'vase', 'scissors',
'teddy bear', 'hair drier', 'toothbrush','london','paris','new york','tour de france']
class_names_arg = ', '.join(class_names)
response = google_images_download.googleimagesdownload()
args = {"keywords":class_names_arg, "limit":100,"print_urls":True}
absolute_image_paths, errors, image_urls = response.download(args)
# print(image_urls)
# temporarily place into .csv
import pandas as pd
try:
# iterate over dict items b/c arrays of different length
df = pd.DataFrame({ key:pd.Series(value) for key, value in image_urls.items() })
df.to_csv('scrape_urls.csv')
except:
print('could not build build df/csv using image_url.items()')
return df
<file_sep>from flask import Flask, render_template, request, jsonify, redirect
import pymongo
import os
from mongoFetch import mongoFetchClasses
app = Flask(__name__)
stored_object = {}
output_images = []
target = 'static/images'
output_dir = 'static/output'
if not os.path.isdir(target):
os.mkdir(target)
else:
print("Couldn't create upload directory: {}".format(target))
if not os.path.isdir(output_dir):
os.mkdir(output_dir)
else:
print("Couldn't create output directory: {}".format(output_dir))
@app.route("/")
def homepage():
# store mongo data in gloval variable
global stored_object
# fetch all db data and place into api
stored_object = mongoFetchClasses()
return render_template('index.html')
@app.route("/fetch/api")
def fetchapi():
# return mongoDB as JSON data
return jsonify(stored_object)
@app.route("/uploadfiles")
def uploadfiles():
output_images = ['static/output/' + img for img in os.listdir('static/output/')]
return render_template('upload.html', output_images=output_images)
@app.route('/uploadfiles/saveimages', methods=["POST"])
def saveimages():
for upload in request.files.getlist("file"):
print("{} is the filename".format(upload.filename))
filename = upload.filename
destination = "/".join([target, filename])
print("Accept incoming file:", filename)
image_path = 'static/images/' + upload.filename
# print("Save it to:", destination)
upload.save(destination)
file_name_root = upload.filename.split('.')[0]
# create an output file name
file_output_name = file_name_root + '_output.png'
# append file output name for later
output_images.append(file_output_name)
# run ML function
image, output_image_matrix, classes_detected = maskImage(destination, ('website/static/output/' + file_output_name))
os.remove(destination)
# redirect to home page
return redirect("/uploadfiles")
if __name__ == "__main__":
app.run(debug=False)<file_sep># Project_3
Objective:
Image Recognition using Mask_RCNN Machine Learning Algoirthm.
Build an interactive UI/application using Python Flask/Pandas/Numpy, MongoDB, HTML, CSS, Javascript, MS Coco database, Mask_RCNN model, Heroku.
Steps:
a. Upload the image in the UI, and display the raw image in the UI
b. Store the raw image in the DB
c. Use Mask_RCNN model to process and identify the object using MS Coco database and determine if the image can be identified (classified) and masked; otherwise pass a message stating 'Image cannot be recognized'
d. Display the processed/Masked image in the UI
e. Possibly Store the Masked image in Mongo DB (possibly constrained by free space available for use on MongoDB )
f. Upload videos and split the video into multiple frames/photos
g. Repeat steps a-e above
<!--Determining the Relationship betweent Uber Demand and the Weather (NYC)-->
<!--This project aims to determine the relationship between Uber ride demand and the historical weather. The team predicts that riderhsip will increase dramatically under less-than-ideal conditions (rain, snow).-->
<!--Reach Goal: Compare demand and weather to average subway ridership and locations per day-->
<!--## Datasets-->
<!--* [Kaggle](https://www.kaggle.com/fivethirtyeight/uber-pickups-in-new-york-city#uber-raw-data-jun14.csv)-->
<!--* [Weather](https://openweathermap.org/history)-->
<!--* [MTA Ridership (bonus)](http://web.mta.info/nyct/facts/ridership/ridership_sub.htm)-->
<!-- * [Weekly Turnstile Data](http://web.mta.info/developers/turnstile.html)--><file_sep>from maskImage import maskImage
from maskImage import startModel
from mongoPush import db_push
from googleImageScrape import scrape_images
import os
import pandas as pd
from natsort import natsorted
model = startModel()
# scrape 100 images of each type
df_urls = scrape_images()
# df_urls = pd.read_csv('scrape_urls.csv')
main_directory = os.getcwd()
downloads = os.path.join(main_directory, 'downloads')
for _, d, _ in os.walk(downloads):
for folder in sorted(d):
print(folder)
current_folder = os.path.join(downloads, folder)
print(current_folder)
for _, _, f in os.walk(current_folder):
for file in natsorted(f):
try:
print(file)
# use this index embedded in the file name to prevent conflict with random, inexplicable nan values
index = int(file.split('.')[0]) - 1
current_file = os.path.join(current_folder, file)
# locate url in df based on folder name and index
img_url = df_urls[folder].iloc[index]
print(img_url)
# return info from function
classes_detected, scores, mask_locations, class_percentages, image_shape = maskImage(current_file, model)
# pass into db
db_push(classes_detected, scores, mask_locations, img_url, class_percentages, image_shape)
except:
print('error thrown for file: ', file)
<file_sep>import pymongo
def mongoFetchClasses():
client = pymongo.MongoClient("mongodb+srv://team123:<EMAIL>/test?retryWrites=true")
db = client.test
collection = db.image_store
# retrieve object based on given class
stored_objects = collection.find() #{'classes_detected': class_to_lookup}
# remove the id since it is not json serializable
cleaned_objects = []
for obj in stored_objects:
obj.pop('_id')
cleaned_objects.append(obj)
# grab image url
# image_url = stored_object['image_url']
# print(image_url)
# return url for use in the html page
return cleaned_objects
<file_sep>gunicorn==19.9.0
pymongo==3.7.2
Flask==1.0.2
dnspython==1.16.0
<file_sep>import cv2
import numpy as np
def colorPercentage(img):
# input image as matrix
imgHSV = cv2.cvtColor(img, cv2.COLOR_RGB2HSV)
red = [0, 25]
orange = [25, 30]
yellow = [30, 40]
green = [40, 80]
blue = [80, 130]
purple = [130, 179]
colors = [blue, red, green, yellow, orange, purple]
colorStrings = ["blue", "red", "green", "yellow", "orange", "purple"]
cp = {}
ranges = []
for i in range(len(colors)):
ranges.append([np.array([colors[i][0], 20, 40]), np.array([colors[i][1], 255, 255])])
mask = cv2.inRange(imgHSV, ranges[i][0], ranges[i][1])
percentage = round(np.sum(mask == 255) / (mask.shape[0] * mask.shape[1]) * 100)
cp[colorStrings[i]] = percentage
whiteRange = [np.array([0, 0, 240]), np.array([255, 20, 255])]
blackRange = [np.array([0, 0, 0]), np.array([255, 255, 39])]
bw = [whiteRange, blackRange]
bwStrings = ["white", "black"]
for i in range(len(bw)):
ranges.append(bw[i])
mask = cv2.inRange(imgHSV, ranges[i+6][0], ranges[i+6][1])
percentage = round(np.sum(mask == 255) / (mask.shape[0] * mask.shape[1]) * 100)
print(bwStrings[i], percentage)
cp[bwStrings[i]] = percentage
return cp<file_sep>def db_push(classes_detected, scores, mask_locations, image_url, color_percentages, image_shape):
import pymongo
import pandas as pd
import datetime
client = pymongo.MongoClient("mongodb+srv://ecalzolaio:<EMAIL>/test?retryWrites=true")
db = client.test
collection = db.image_store
# image_rescaled = image_rescaled.tolist()
mask_locations = mask_locations.tolist()
to_insert = {
# "image_rescaled": image_rescaled,
"classes_detected": classes_detected,
"scores":[float(score) for score in scores],
"mask_locations": mask_locations,
"image_url": image_url,
"color_percentages": color_percentages,
"image_shape": image_shape,
"date": datetime.datetime.now()
}
collection.insert_one(to_insert)
# Issue the serverStatus command and print the results
# serverStatusResult=db.command("serverStatus")
# pprint(serverStatusResult)<file_sep>import cv2
import numpy as np
import os
import sys
from maskImage import maskImage, startModel
def videoToImage(file_name):
model = startModel()
APP_ROOT = os.path.abspath("Upload_Scrape_Mongo_Push/")
vid_image_dir = os.path.join(APP_ROOT,'static','videoToImage')
print(vid_image_dir)
if not os.path.isdir(vid_image_dir):
os.mkdir(vid_image_dir)
else:
print("Couldn't create raw directory: {}".format(vid_image_dir))
processed_video_dir = os.path.join(APP_ROOT,'static', 'processedVideoImage')
if not os.path.isdir(processed_video_dir):
os.mkdir(processed_video_dir)
else:
print("Couldn't create crop directory: {}".format(processed_video_dir))
# def videoToFrames(video, everyNthFrame=30)
# cap = cv2.VideoCapture(video)
file_location = os.path.join(vid_image_dir, file_name)
cap = cv2.VideoCapture(file_location)
frameList = []
currentFrame = 1
while(True):
# Capture frame-by-frame
ret, frame = cap.read()
if ret == False:
break
# Saves every Nth frame as jpg file
# if currentFrame % everyNthFrame == 0:
if currentFrame % 30 == 0:
name = str(currentFrame) + '.jpg'
image_location = os.path.join(vid_image_dir, name)
print ('Creating...' + name)
cv2.imwrite(image_location, frame)
output_name = os.path.join(processed_video_dir, name)
maskImage(image_location, model, output_name)
# frameList.append(frame)
# To stop duplicate images
currentFrame += 1
# return frameList
| 373ba962e18951c832887f9a634a74995c3facd1 | [
"JavaScript",
"Python",
"Text",
"Markdown"
] | 11 | JavaScript | vladknezdata/imageRecognition | 74e8c379250c17570c106104b9432275822e7ebf | 7e63002c1a6024fc8ed62587fd40dd7a4e066137 | |
refs/heads/master | <file_sep>package sg.edu.rp.c346.id20016584.c346_assignment;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.text.Editable;
import android.text.TextWatcher;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ArrayAdapter;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ListView;
import android.widget.Spinner;
import android.widget.Toast;
import java.util.ArrayList;
import java.util.Calendar;
import java.util.Collections;
public class ItemListActivity extends AppCompatActivity {
EditText etproduct, etindex, etexpiry,etspin;
Button btnadd, btnupdate, btndel;
Spinner spin;
ListView lv;
ArrayList<String> alproduct;
ArrayAdapter<String> aaproduct;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_item_list);
etproduct=findViewById(R.id.Product);
etindex=findViewById(R.id.index);
etexpiry=findViewById(R.id.expiry);
etspin=findViewById(R.id.DateRange);
btnadd=findViewById(R.id.buttonAdd);
btndel=findViewById(R.id.buttonDelete);
btnupdate=findViewById(R.id.buttonUpdate);
spin=findViewById(R.id.spinner);
lv=findViewById(R.id.list);
alproduct=new ArrayList<>();
alproduct.add("Expires 2022-8-6 Logi mouse");
alproduct.add("Expires 2022-10-10 Razor keyboard");
alproduct.add("Expires 2021-12-8 Razor headset");
alproduct.add("Expires 2021-8-23 Portable charger");
alproduct.add("Expires 2022-3-11 JBL speaker");
aaproduct=new ArrayAdapter<>(this, android.R.layout.simple_list_item_1, alproduct);
lv.setAdapter(aaproduct);
btnadd.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String strP= etproduct.getText().toString();
String strE= etexpiry.getText().toString();
alproduct.add("Expires "+strE+" "+strP);
Collections.sort(alproduct, String.CASE_INSENSITIVE_ORDER);
aaproduct.notifyDataSetChanged();
}
});
btnupdate.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String strP= etproduct.getText().toString();
String strE= etexpiry.getText().toString();
int index=Integer.parseInt(etindex.getText().toString());
alproduct.set(index, "Expires "+strE+" "+strP);
Collections.sort(alproduct, String.CASE_INSENSITIVE_ORDER);
aaproduct.notifyDataSetChanged();
}
});
btndel.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
int index=Integer.parseInt(etindex.getText().toString());
alproduct.remove(index);
aaproduct.notifyDataSetChanged();
}
});
//ERROR:THE CODE CANNOT RUN WITH THE SPINNER CODE BELOW
spin.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
switch (position){
case 0:
int month= Calendar.getInstance().get(Calendar.MONTH)+1;
int year= Calendar.getInstance().get(Calendar.YEAR);
String ym=year+"-"+month;
etspin.setText(ym);
case 1:
month= Calendar.getInstance().get(Calendar.MONTH)+3;
year= Calendar.getInstance().get(Calendar.YEAR);
ym=year+"-"+month;
etspin.setText(ym);
case 2:
month= Calendar.getInstance().get(Calendar.MONTH)+6;
year= Calendar.getInstance().get(Calendar.YEAR);
ym=year+"-"+month;
etspin.setText(ym);
}
}
});
etspin.addTextChangedListener(new TextWatcher() {
@Override
public void beforeTextChanged(CharSequence s, int start, int count, int after) {
}
@Override
public void onTextChanged(CharSequence s, int start, int before, int count) {
aaproduct.getFilter().filter(s);
}
@Override
public void afterTextChanged(Editable s) {
}
});
}
}<file_sep>rootProject.name = "c346_assignment"
include ':app'
| dad48cf65283089591e8b2f2166bd4b0bdc5bfb5 | [
"Java",
"Gradle"
] | 2 | Java | Jaden-Ng/c346_assignment | eeefe6ee3d2cd33a6140c8b4379e41ef12827f2d | 8bdc74778bffc09315358488da33eb0e636034dd | |
refs/heads/master | <file_sep>import numpy as np
x = np.array([(1,2.,'Hello'), (2,3.,"world")],dtype = [('foo', 'i4'),('bar', 'f4'), ('baz', 'S10')])
print(x[1])
y = x['bar']
print(y)
y[:] = 2*y
print(y) #4,6
print(x) #(1, 4., b'Hello') (2, 6., b'world')
x[1] = (-1,-1., "Master")
print(x)
x = np.zeros(3, dtype='3int8, float32, (2,3)float64')
print(x)
<file_sep>import torch
import torchvision
import torchvision.datasets as dset
import torchvision.transforms as transforms
from torch.autograd import Variable
import torch.nn as nn
import torch.nn.functional as F
import torch.optim as optim
class LeNet(nn.Module):
def __init__(self):
super(LeNet, self).__init__()
self.conv1 = nn.Conv2d(1, 6, 5)
self.pool = nn.MaxPool2d(2, 2)
self.conv2 = nn.Conv2d(6, 16, 5)
self.fc1 = nn.Linear(4*4*16, 120)
self.fc2 = nn.Linear(120, 84)
self.fc3 = nn.Linear(84, 10)
self.criterion = nn.CrossEntropyLoss()
def forward(self, x,target):
x = self.pool(F.relu(self.conv1(x)))
x = self.pool(F.relu(self.conv2(x)))
#print(x.size())
x = x.view(-1, 4*4*16)
x = F.relu(self.fc1(x))
x = F.relu(self.fc2(x))
x = F.relu(self.fc3(x))
loss = self.criterion(x,target)
return x,loss
def name(self):
return 'LeNet-5'
transform = transforms.Compose(
[transforms.ToTensor(),
transforms.Normalize((0.1307,), (0.3081,))])
batch_size = 128
kwargs = {'num_workers': 1, 'pin_memory': True}
trainset = dset.MNIST(root='MNIST', train=True, transform=transform, download=True)
train_loader = torch.utils.data.DataLoader(trainset, batch_size = batch_size, shuffle=True, **kwargs)
testset = dset.MNIST(root='MNIST', train=False, transform=transform)
test_loader = torch.utils.data.DataLoader(testset, batch_size=batch_size,shuffle=False, **kwargs)
print ('==>>> total training batch number: {}'.format(len(train_loader)))
print ('==>>> total testing batch number: {}'.format(len(test_loader)))
model = LeNet()
optimizer = optim.SGD(model.parameters(), lr=0.01, momentum=0.9)
for epoch in range(10): # loop over the dataset multiple times
#training
for batch_idx, (data, target) in enumerate(train_loader):
optimizer.zero_grad()
data, target = Variable(data), Variable(target)
_,loss = model(data, target)
loss.backward()
optimizer.step()
if batch_idx % 100 == 0:
print ('==>>> epoch: {}, batch index: {}, train loss: {:.6f}'.format(epoch, batch_idx, loss.data[0]))
#testing
correct_cnt, ave_loss = 0,0
for batch_idx, (data,target) in enumerate(test_loader):
x,target = Variable(data),Variable(target)
score,loss = model(x,target)
_,pred_label = torch.max(score.data,1)
correct_cnt += (pred_label == target.data).sum()
ave_loss += loss.data[0]
accuracy = correct_cnt*1.0/len(test_loader)/batch_size
ave_loss /= len(test_loader)
print ('==>>> epoch: {}, test loss: {:.6f}, accuracy: {:.4f}'.format(epoch, ave_loss, accuracy))
torch.save(model.state_dict(), model.name())
<file_sep>import os
os.system("./svm_learn BNP_test.txt model.txt ")<file_sep>import numpy as np
#Printing Arrays
a = np.arange(6) #일차원 배열
b = np.arange(12).reshape(4,3) #2차원배열
c = np.arange(24).reshape(2,3,4) #3차원배열
A = np.array( [[1,1], [0,1]] )
B = np.array( [[2,0], [3,4]] )
print(A*B) #elementwise product
A.dot(B) #matrix product
np.dot(A, B) #another matrix product
a = np.random.random((2,3))
print(a)
print(a.min())
print(a.max())
b = np.arange(12).reshape(3,4)
print(b.sum(axis=0)) #sum of each column
b.min(axis=1) #min of each row
b.cumsum(axis=1) #cumulative sum along each row
#Universal Function
B = np.arange(3)
np.exp(B)
np.sqrt(B)
C = np.array([2., -1., 4.])
D = np.add(B, C)
#Indexing, Slicing and Iterating
a = np.arange(10)**3 #원소들값 3제곱
#Multidimensional
def f(x,y):
return 10*x+y
b = np.fromfunction(f,(5,4),dtype=int)
b[0:5, 1] # each row in the second column of
b[ : ,1]
b[1:3, : ] # each column in the second and third row of b
for row in b:
print(row)
for element in b.flat: #한원소씩 접근
print(element)
#Stacking together different arrays
a = np.floor(10*np.random.random((2,2)))
print(a)
b = np.floor(10*np.random.random((2,2)))
np.vstack((a,b))
np.hstack((a,b))
np.column_stack((a,b))
#Splitting one array into several smaller ones
a = np.floor(10*np.random.random((2,12)))
np.hsplit(a,3) #split a into 3
np.hsplit(a,(3,4)) # Split a after the third and the fourth column
#Copies and Views
a = np.arange(12)
b = a
b.shape = 3,4
a.shape
#View or Shallow Copy
c = a.view()
c is a #true
c.base is a
#deep copy
d = a.copy()
d is a #false
#Linear Algebra
#Simple Array Operations
a = np.array([[1.0, 2.0], [3.0, 4.0]])
a.transpose()
u = np.eye(2) # unit 2x2 단위 matrix 생성
np.dot (j, j) # matrix product
<file_sep>#############Sequence#################
#1)make whole word list from train.txt, text.txt
#2)make word_cnt_dic based on acq or not AND make tf*idf dictionary => {word1 : 0.xxxx, word2 : 0.xxx, ....}
#3)make input_train, input_test files for svm
#4)run
from nltk.stem.snowball import SnowballStemmer
import re
import math
import copy
def make_stopword_list(f_name):
f = open(f_name)
for stopword in f:
stopword_list = stopword.split(' ')
return stopword_list
#delete stopword(s) in word list
def delete_stopword(word_list, stopword_list):
for i in range(len(stopword_list)):
if stopword_list[i] in word_list:
word_list.remove(stopword_list[i])
return
def UseStemmer(word_list,stemmer_obj):
word_cnt = len(word_list)-2
for i in range(1,word_cnt+1):
word_list[i] = stemmer_obj.stem(word_list[i])
return
def delete_word(complete_dic, word_dic):
acq_word_list = word_dic['acq']
etc_word_list = word_dic['etc']
delete_acq_word = []
delete_etc_word = []
acq_word_cnt_dic = complete_dic['acq']
etc_word_cnt_dic = complete_dic['etc']
#delete from cnt_dic
for word in acq_word_list:
if acq_word_cnt_dic[word] < 5:
delete_acq_word.append(word)
acq_word_cnt_dic.pop(word)
for word in etc_word_list:
if etc_word_cnt_dic[word] < 5:
delete_etc_word.append(word)
etc_word_cnt_dic.pop(word)
#delete form word_list
for word in acq_word_list:
if word in delete_acq_word:
acq_word_list.pop(word)
for word in etc_word_list:
if word in delete_etc_word:
etc_word_list.pop(word)
return
def make_word_dic(file_name, stemmer, stopword_list):
word_dic = {}
f = open(file_name)
for line in f:
line_list = re.split('\t| ', line)
delete_stopword(line_list, stopword_list)
UseStemmer(line_list, stemmer)
if line_list[0] == 'acq':
input_class = line_list[0]
else:
input_class = 'etc'
word_cnt = len(line_list) - 2
# Check input_class is in input_dic
if input_class not in word_dic:
word_dic[input_class] = []
word_list = word_dic[input_class]
for i in range(1, word_cnt + 1):
input_word = line_list[i]
if input_word in word_list:
continue
else:
word_list.append(input_word)
return word_dic
def combine_two_word_list(word_list1, word_list2):
new_word_list = copy.deepcopy(word_list1)
for word2 in word_list2:
if word2 not in new_word_list:
new_word_list.append(word2)
return new_word_list
def make_word_list(complete_word_dic):
acq_word_list = complete_word_dic['acq']
etc_word_list = complete_word_dic['etc']
new_word_list = combine_two_word_list(acq_word_list,etc_word_list)
return new_word_list
def make_output_dictionary(file_name, stemmer, stopword_list ):
output_dic = {}
f = open(file_name)
num_of_doc = 0
# make dictionary => {'acq' : { 'word' : 0, ... } , 'etc' : { 'word' : 0, ... } }
for line in f:
num_of_doc += 1
# line list contains => first element : class , last element : newline
line_list = re.split('\t| ', line)
delete_stopword(line_list, stopword_list)
UseStemmer(line_list, stemmer)
if line_list[0] == 'acq':
input_class = line_list[0]
else:
input_class = 'etc'
word_cnt = len(line_list) - 2
# Check input_class is in input_dic
if input_class not in output_dic:
output_dic[input_class] = {}
word_list = output_dic[input_class]
# make word in class list or increase the count
for i in range(1, word_cnt + 1):
input_word = line_list[i]
if input_word in word_list:
word_list[input_word] += 1
else:
word_list[input_word] = 1
return output_dic,num_of_doc
def calc_all_value(dic,list):
all_num = 0
for temp in list:
all_num += dic[temp]
return all_num
def calc_tf_idf(word_cnt,all_acq_word_num,num_of_doc,doc_cnt):
term_freq = word_cnt/all_acq_word_num
inv_doc_freq = math.log(num_of_doc/doc_cnt)
result = term_freq * inv_doc_freq
return result
def make_doc_cnt_dic(file_name, stemmer, stopword_list,complete_word_list):
word_doc_cnt = {}
for word in complete_word_list:
word_doc_cnt[word] = 0
f = open(file_name)
for line in f:
line_list = re.split('\t| ', line)
delete_stopword(line_list, stopword_list)
UseStemmer(line_list, stemmer)
for word in complete_word_list:
if word in line_list:
word_doc_cnt[word] += 1
return word_doc_cnt
def make_tf_idf_dic(complete_dic,complete_word_dic,all_word_list,num_of_doc,doc_cnt_dic):
output_dic = {}
acq_cnt_dic = complete_dic['acq']
acq_word_list = complete_word_dic['acq']
all_acq_word_num = calc_all_value(acq_cnt_dic,acq_word_list)
for word in all_word_list:
if word in acq_word_list:
output_dic[word] = calc_tf_idf(acq_cnt_dic[word],all_acq_word_num,num_of_doc,doc_cnt_dic[word])
else:
output_dic[word] = 0
return output_dic
def make_svm_input(input_file_name,stopword_list,stemmer,output_file_name,tf_idf_dic,all_word_list):
input_file = open(input_file_name)
output_file = open(output_file_name,'w')
for line in input_file:
# line list contains => first element : class , last element : newline
output_str = ''
line_list = re.split('\t| ', line)
delete_stopword(line_list, stopword_list)
UseStemmer(line_list, stemmer)
word_cnt = len(line_list) - 2
#extract words from line_list AND make sorted list ascending order
temp_list = line_list[1:word_cnt+1]
sorted_list = []
for word in all_word_list:
if word in temp_list:
sorted_list.append(word)
#make output stirng
if line_list[0] == 'acq':
output_str += '1'
else:
output_str += '-1'
for word in sorted_list:
index = all_word_list.index(word)+1
if tf_idf_dic[word] == 0:
continue
output_str += ' '+str(index) + ':' + str(tf_idf_dic[word])
output_str += '\n'
output_file.write(output_str)
return
##################################MAIN###################################
#make stemmer object and stopword list
stemmer = SnowballStemmer("english", ignore_stopwords=False)
stop_word_f_name = 'stopword.txt'
stopword_list = make_stopword_list(stop_word_f_name)
#make word list from train set, tf*idf dictionay => {word1 : 0.xxxx, word2 : 0.xxxx, word3 : 0.xxxx, ....}
train_input_f_name = 'train.txt'
complete_output_dic,num_of_doc = make_output_dictionary(train_input_f_name,stemmer,stopword_list)
complete_word_dic = make_word_dic(train_input_f_name,stemmer,stopword_list)
complete_word_list = make_word_list(complete_word_dic)
doc_cnt_dic = make_doc_cnt_dic(train_input_f_name,stemmer,stopword_list,complete_word_list)
tf_idf_dic = make_tf_idf_dic(complete_output_dic,complete_word_dic,complete_word_list,num_of_doc,doc_cnt_dic)
#make output files
test_input_f_name = 'test.txt'
train_output_f_name = 'BNP_train.txt'
test_output_f_name = 'BNP_test.txt'
make_svm_input(train_input_f_name,stopword_list,stemmer,train_output_f_name,tf_idf_dic,complete_word_list)
make_svm_input(test_input_f_name,stopword_list,stemmer,test_output_f_name,tf_idf_dic,complete_word_list)
<file_sep>import numpy as np
x = np.array([2,3,1,0])
x = np.array([2, 3, 1, 0])
x = np.array([[1,2.0],[0,0],(1+1j,3.)]) #허수 값을로 이루어진 배열
print(x)
x = np.array([[ 1.+0.j, 2.+0.j], [ 0.+0.j, 0.+0.j], [ 1.+1.j, 3.+0.j]]) #5행과 같은표현
print(x)
np.arange(10) #array([0, 1, 2, 3, 4, 5, 6, 7, 8, 9])
np.arange(2, 10, dtype=np.float) #array([ 2., 3., 4., 5., 6., 7., 8., 9.])
np.arange(2, 3, 0.1) #array([ 2. , 2.1, 2.2, 2.3, 2.4, 2.5, 2.6, 2.7, 2.8, 2.9]) , #2이상 3미만인 수들중 0.1간격으로 출력
np.linspace(1., 4., 6) #array([ 1. , 1.6, 2.2, 2.8, 3.4, 4. ]) , 1이상 4이하인 수중 6개의 수를 선택
<file_sep>class Node:
data = ''
child_dic = {}
attr_name_list = []
def __init__(self, attr_name = None, attr_list = None):
if attr_name is None:
return
self.data = attr_name
if attr_list is None:
return
for temp in attr_list:
self.child_dic[temp] = Node()
self.attr_name_list.append(temp)
def print_child_dic(self):
print(self.child_dic)
def get_child_dic(self):
return self.child_dic
def print_nodes(self):
if self.data == 'empty':
print('empty terminal node')
return
print(self.data)
if self.data is 'e' or self.data is 'p': #terminal node이면 return
return
test_node = Node('abcd')
test_node = Node()
print(test_node.data)<file_sep>training_data = open('xxx.data.txt','r')
for line in training_data:
temp1 = line.split(',')
if (temp1[22].strip('\n') == 'p' or temp1[22].strip('\n') == 'u'):
print(temp1)
print('없음')
<file_sep>
def func_word(result_dic, words):
for word in words:
if word in result_dic:
result_dic[word]+=1
else:
result_dic[word] = 1
def find_max_in_dic(result_dic):
max = -1
str = ''
result_str = []
result_str2 = []
for key in result_dic:
if result_dic[key] > max:
max = result_dic[key]
for key in result_dic:
if result_dic[key] == max:
result_str.append(key)
result_str2.append(result_dic[key])
return result_str, result_str2
file_name = input('파일명을 입력하세요 : ')
file_obj = open(file_name, 'r')
list = []
word_cnt = 0
line_cnt = 0
word_dic = {}
word_cnt_dic = {}
for line in file_obj:
temp_word_list = line.split()
func_word(word_dic, temp_word_list )
word_cnt += len(temp_word_list)
line_cnt += 1
word_cnt_dic[line_cnt] = len(temp_word_list)
print('단어수 : ' + str(word_cnt))
print('줄수 : ' + str(line_cnt))
max_word_list, temp = find_max_in_dic(word_dic)
max_word_str= ''.join(max_word_list)
print('가장 많이 쓰인 단어 : ' + max_word_str)
temp_str1, temp_str2 = find_max_in_dic(word_cnt_dic)
max_line_num = ', '.join(str(x) for x in temp_str1)
max_word_num = ', '.join(str(x) for x in temp_str2)
print('단어가 가장 많은 라인 : ' + max_line_num)
print('단어가 가장 많이 포함된 라인에서의 단어수 : ' + max_word_num)<file_sep>import numpy as np
from scipy import linalg
import matplotlib.pyplot as plt
#numpy.matrix vs 2D numpy.ndarray
A = np.mat('[1 2;3 4]')
print(A)
print(A.I) #A의 역행렬 출력
b = np.mat('[5 6]')
print(b.T) #B의 transpose 행렬
print(A*b.T)
A = np.array([[1,2], [3,4]])
print(A)
print(linalg.inv(A)) #scipy모듈로 역행렬 구하기
b = np.array([[5,6]]) #2D array
#finding inverse
A = np.array([[1,3,5], [2,5,1], [2,3,8]])
print(A)
print(linalg.inv(A))
print(A.dot(linalg.inv(A)))
#solving linear system
A = np.array([[1, 2], [3, 4]]) #x+2y = 5 와 3x+4y = 6 연립방정식 해구하기
b = np.array([[5], [6]])
linalg.inv(A).dot(b)
A.dot(linalg.inv(A).dot(b)) - b #0이 되는지 확인
np.linalg.solve(A, b) #해를 구하는 더 빠른 방법
A.dot(np.linalg.solve(A, b)) - b
#Finding Determinant
A = np.array([[1,2],[-3,4]])
linalg.det(A) #determinant 구하기
#computing norms
A=np.array([[1,2],[3,4]])
linalg.norm(A)
#Solving linear least-squares problems and pseudo-inverses
c1, c2 = 5.0, 2.0
i = np.r_[1:11]
xi = 0.1*i
yi = c1*np.exp(-xi) + c2*xi
zi = yi + 0.05 * np.max(yi) * np.random.randn(len(yi))
A = np.c_[np.exp(-xi)[:, np.newaxis], xi[:, np.newaxis]]
c, resid, rank, sigma = linalg.lstsq(A, zi)
xi2 = np.r_[0.1:1.0:100j]
yi2 = c[0]*np.exp(-xi2) + c[1]*xi2
plt.plot(xi,zi,'x',xi2,yi2)
plt.axis([0,1.1,3.0,5.5])
plt.xlabel('$x_i$')
plt.title('Data fitting with linalg.lstsq')
plt.show()<file_sep>from __future__ import unicode_literals, print_function
import pycrfsuite
def index2feature(sent, i, offset): #index 정보를 첨가하여 string 반환
word, tag = sent[i + offset]
if offset < 0:
sign = ''
else:
sign = '+'
return '{}{}:word={}'.format(sign, offset, word)
def word2features(sent, i): #현재 형태소 앞에 2개와 뒤에 2개정보를 첨가하여 리스트로 반환
L = len(sent)
word, tag = sent[i]
features = ['bias']
features.append(index2feature(sent, i, 0))
if i > 1:
features.append(index2feature(sent, i, -2))
if i > 0:
features.append(index2feature(sent, i, -1))
else:
features.append('bos')
if i < L - 2:
features.append(index2feature(sent, i, 2))
if i < L - 1:
features.append(index2feature(sent, i, 1))
else:
features.append('eos')
return features
def sent2tags(sent):
return [tag for word, tag in sent]
def sent2features(sent):
return [word2features(sent, i) for i in range(len(sent))]
def inputToList(f_name,true_list): #train.txt를 읽어서 정답리스트와 [형태소,태그] 리스트 생성
input_f = open(f_name, 'r', encoding='utf-8')
total_sentence = []
for line in input_f: #한줄씩 읽기
if (line == '\n'): #개행이면 무시
continue
word,tags = line.split(' ')
tags = tags.rstrip() # \n제거
#print(tags)
if '+' in tags: #'+'문자 기준으로 나누기
tags = tags.split('+')
sentences1 = []
temp_sentence = []
for temp2 in tags:
sentence = []
if temp2[0] == '/': #첫문자가 '/' 인경우
temp_word = '/'
temp_tag = temp2[2:]
else:
temp_word, temp_tag = temp2.split('/')
sentence.append(temp_word)
temp_sentence.append(temp_tag)
sentence.append(temp_tag)
sentences1.append(sentence)
total_sentence.append(sentences1)
true_list.append(temp_sentence)
else: #'+' 없는경우(그 자체가 하나의 형태소인경우)
sentence = []
sentences1 = []
temp_sentence = []
if tags[0] == '/': #첫문자가 '/' 인경우
temp_word = '/'
temp_tag = tags[2:]
else:
temp_word, temp_tag = tags.split('/')
temp_sentence.append(temp_tag)
sentence.append(temp_word)
sentence.append(temp_tag)
sentences1.append(sentence)
total_sentence.append(sentences1)
true_list.append(temp_sentence)
return total_sentence #전체 [형태소, 태그] 리스트 반환
def test_result(list1,list2):
print('******test******')
all_num = len(list1) #전체 항목갯수
true_num = 0 #맞은 갯수
for i in range(all_num):
if list1[i] == list2[i]: #두 태깅 결과가 일치
true_num += 1 #맞은 갯수 카운트 +1
success_rate = true_num/all_num #정답률 계산
print('Success Rate : '+str(success_rate))
true_list = [] #정답을 저장할 리스트
train_sents = inputToList('train.txt',true_list) #train.txt를 읽어서 [형태소,태그]들의 리스트로 변환
print(train_sents)
train_x = [sent2features(sent) for sent in train_sents] #[형태소,태그] 리스트를 가지고 형태소간의 index 정보를 추가한 리스트로 변환
print(train_x)
train_y = [sent2tags(sent) for sent in train_sents] #[형태소, 태그] 리스트를 가지고 tag정보들 반환
print(train_y)
trainer = pycrfsuite.Trainer() #학습객체 생성
for x, y in zip(train_x, train_y):
trainer.append(x, y) #해당 형태소의 앞뒤의 형태소정보와 태그 정보를 가지고 학습
trainer.train('model.crfsuite')
tagger = pycrfsuite.Tagger()
tagger.open('model.crfsuite')
true_list = [] #정답을 저장할 리스트
test_sents = inputToList('train.txt',true_list) #train.txt를 읽어서 [형태소,태그]들의 리스트로 변환
test_x = [sent2features(sent) for sent in test_sents] #[형태소,태그] 리스트를 가지고 형태소간의 index 정보를 추가한 리스트로 변환
test_y = [sent2tags(sent) for sent in test_sents] #[형태소, 태그] 리스트를 가지고 tag정보들 반환
pred_y = [tagger.tag(x) for x in test_x] #예측값 도출
print(pred_y)
test_result(true_list,pred_y) #정답과 예측값을 비교하는함수<file_sep>from nltk.stem.snowball import SnowballStemmer
import re
import math
import operator
#parameter => file name, stemmer obj, stopword_list
#return complete_dictionary,class name list, number of class, class count dictionary
def make_dictionary(file_name, stemmer, stopword_list ):
input_dic = {}
cnt_in_class = {}
class_name_list = []
f = open(file_name)
num_of_class = 0
num_of_training_data = 0
# make dictionary => {'earn' : { 'word' : 0, ... } , ... }
for line in f:
num_of_training_data += 1
# line list contains => first element : class , last element : newline
line_list = re.split('\t| ', line)
delete_stopword(line_list, stopword_list)
UseStemmer(line_list, stemmer)
input_class = line_list[0]
word_cnt = len(line_list) - 2
# Check input_class is in input_dic
if input_class not in input_dic:
input_dic[input_class] = {}
num_of_class+=1
cnt_in_class[input_class] = 1
class_name_list.append(input_class)
else:
cnt_in_class[input_class] += 1
word_list = input_dic[input_class]
# make word in class list or increase the count
for i in range(1, word_cnt + 1):
input_word = line_list[i]
if input_word in word_list:
word_list[input_word] += 1
else:
word_list[input_word] = 1
return input_dic, cnt_in_class,num_of_class,class_name_list, num_of_training_data
#parameter => file_name, complete dictionary form training set, class name lsit, class probability list, stemmer obj, stopword list
#return => number of test data, success rate from test set
def exec_test(file_name,input_dic,class_name_list,class_prob_dic,stemmer,stopword_list):
f = open(file_name)
all_num = 0
true_num = 0
num_of_test_data = 0
for line in f:
num_of_test_data += 1
all_num += 1
# line list contains => first element : class , last element : newline
line_list = re.split('\t| ', line)
delete_stopword(line_list, stopword_list)
UseStemmer(line_list, stemmer)
true_class = line_list[0]
answer = calc_answer(input_dic, class_name_list, class_prob_dic, line_list)
if answer == true_class:
true_num += 1
success_rate = true_num/all_num
return num_of_test_data,success_rate
#delete stopword(s) in word list
def delete_stopword(word_list, stopword_list):
for i in range(len(stopword_list)):
if stopword_list[i] in word_list:
word_list.remove(stopword_list[i])
return
def UseStemmer(word_list,stemmer_obj):
word_cnt = len(word_list)-2
for i in range(1,word_cnt+1):
word_list[i] = stemmer_obj.stem(word_list[i])
return
def make_class_prob_dic(cnt_class_dic, class_list):
class_prob_dic = {}
num_of_input = sum(cnt_class_dic.values())
for class_name in class_list:
class_prob_dic[class_name] = cnt_class_dic[class_name]/num_of_input
return class_prob_dic
def calc_BayesClassifier(word_cnt_info, class_name, class_prob_dic, input_list):
input_word_cnt = len(input_list) - 2
log_sum = 0
temp_word_cnt_dic = {}
temp_word_list = []
zero_cnt = 0
#count zero count in input list if word is not in complete_word_dic, save word and word count to temp_dic
for i in range(1,input_word_cnt+1):
temp_word_list.append(input_list[i]) #append word
if input_list[i] in word_cnt_info.keys():
temp_word_cnt_dic[input_list[i]] = word_cnt_info[input_list[i]]
else:
temp_word_cnt_dic[input_list[i]] = 1
zero_cnt += 1
#calculate log summation
for temp_word in temp_word_list:
numerator = temp_word_cnt_dic[temp_word]
denominator = sum(word_cnt_info.values()) + zero_cnt #분모에 zero개수 만큼 더해준다
log_sum += math.log10(numerator/denominator)
result = math.log10(class_prob_dic[class_name]) + log_sum
return result
def calc_answer(complete_word_dic, class_name_list, class_prob_dic, input_list):
BayesClassifier_dic = {}
for class_name in class_name_list:
BayesClassifier_dic[class_name] = calc_BayesClassifier(complete_word_dic[class_name],class_name,class_prob_dic,input_list)
answer = max(BayesClassifier_dic.items(), key=operator.itemgetter(1))[0]
return answer
def make_stopword_list(f_name):
f = open(f_name)
for stopword in f:
stopword_list = stopword.split(' ')
return stopword_list
###################################MAIN###################################
#make stemmer object and stopword list
stemmer = SnowballStemmer("english", ignore_stopwords=False)
f_name = 'stopword.txt'
stopword_list = make_stopword_list(f_name)
######training#####
f_name = 'train.txt'
input_dic,cnt_in_class,num_of_class,class_name_list,num_of_training_data = make_dictionary(f_name,stemmer,stopword_list)
class_prob_dic = make_class_prob_dic(cnt_in_class,class_name_list)
######testing#####
f_name = 'test.txt'
num_of_test_data, success_rate = exec_test(f_name,input_dic,class_name_list,class_prob_dic,stemmer,stopword_list)
print('##############test################')
print('num of training data : '+str(num_of_training_data))
print('num of test data : '+str(num_of_test_data))
print('Success Rate : ' + str(success_rate))
<file_sep>import numpy as np
x = np.arange(10) #size가 10인 배열생성
print(x[2]) #2
print(x[-2]) #8
x.shape = (2,5) #이제 x는 2치원배열이됨
print(x[1,3]) #8
print(x[1,-1]) #9
print(x[0])
x = np.arange(10) #size 10인 배열생성
print(x[2:5]) #2,3,4
print(x[1:7:2]) #1,3,5
y = np.arange(35).reshape(5,7)
print(y[1:5:2, ::3]) #1,3행에 3열 간격으로 선택하여 배열 구성 => [ [ 7 10 13 ] [ 21 24 27 ] ]
x = np.arange(10,1,-1)
print(x) #array([10, 9, 8, 7, 6, 5, 4, 3, 2])
temp = x[np.array([3, 3, 1, 8])]
print(temp) #array([7, 7, 9, 2])
x[np.array([3,3,-3,8])] #array([7, 7, 4, 2])
temp = y[np.array([0,2,4]), np.array([0,1,2])]
print(temp) # y[0,2], y[2,1], y[4,2]
temp = y[np.array([0,2,4]),1]
print(temp) # y[0,1], y[2,1], y[4,1]
temp = y[np.array([0,2,4])]
print(temp) # 0행,2행,4행 원소들 출력
#Bollean or "mask" index arrays
b = y>20
temp = y[b]
print(temp) #20보다 큰 원소들 모두 출력
x = np.arange(30).reshape(2,3,5) #0~29까지의 원소로 3차원 행렬 생성
print(x)
b = np.array([[True,True,False], [False,True, True]])
print(x[b]) #[x[0][0].x[0][1],x[1][1],x[1][2] 원소출력
#Structural indexing tools
print(y.shape) # (5,7)
temp = y[:,np.newaxis,:].shape
print(temp) #(5,1,7)
x = np.arange(10)
x[2:7] = 1
print(x)
x[2:7] = np.arange(5)
x = np.arange(0,50,10) #0,10,20,30,40
x[np.array([1,1,3,1])] += 1
print(x) # 0,11,20,31,40 =>1은 한번만 가산됨
<file_sep># 경북대학교 인공지능 AI 2017 봄학기 수업과제
## 1. decision tree
22개의 attribute로 판단하는 독버섯과 정상버섯을 판단하는 decision tree를 구현
## 2. MLP:Perceptron
MNIST를 판단하는 single-layer perceptron, multi-layer perceptron 구현
## 3. MNIST_LeNet5,MNIST_rnn
PyTorch로 LeNet-5 convolution neural network 구현 torchvision을 이용하여 MNIST 데이터셋을 import하여 학습 및 테스트
PyTorch로 RNN 구현보고서 작성 및 MNIST 데이터셋을 import하여 학습및 테스트
## 4. bayes_classifier
Naive Bayes로 reuters 기사를 8개의 클래스로 분류하는 분류기 구현
## 5. svm,adaboost
SVM으로 reuters 기사를 acq클래스인지 아닌지 분류하는 모델 생성및 테스트
기존의 svm 결과에서 틀린부분과 맞는부분을 바탕으로 가중치를 변경하여 학습시키는 adaboost 구현
## 6. korean_spacing_predictor
CRF(Conditional Random Field)를 이용하여 한국어 띄어쓰기 프로그램 구현
## 7. korean_lexical_predictor(incomplete)
CRF를 이용하여 한국어 형태소 분류기 구현
<file_sep>import random
def calc_output(x_list,w_list):
result = 0
for i in range(65):
result = result + x_list[i]*w_list[i]
if result > 0:
result = 1
else:
result = 0
return result
def calc_output2(x_list,w_list):
result = 0
for i in range(65):
result = result + x_list[i]*w_list[i]
return result
def change_w_list(w_list,x_list,learning_rate,target,output):
for i in range(len(w_list)):
w_list[i] = w_list[i] + learning_rate*(target-output)*x_list[i]
return
learning_rate = 0.1
#perceptron 초기화
percept_dic = {0 : [], 1 : [], 2 : [], 3 : [], 4 : [], 5 : [], 6 : [], 7 : [], 8 : [], 9 : []}
for i in range(65):
percept_dic[0].append(random.uniform(0, 1))
percept_dic[1].append(random.uniform(0, 1))
percept_dic[2].append(random.uniform(0, 1))
percept_dic[3].append(random.uniform(0, 1))
percept_dic[4].append(random.uniform(0, 1))
percept_dic[5].append(random.uniform(0, 1))
percept_dic[6].append(random.uniform(0, 1))
percept_dic[7].append(random.uniform(0, 1))
percept_dic[8].append(random.uniform(0, 1))
percept_dic[9].append(random.uniform(0, 1))
#training_data = open('a.txt','r')
for temp in range(3):
training_data = open('optdigits.tra', 'r')
for line in training_data:
x_list = [1]
temp_list = line.split(',')
for i in range(64):
x_list.append(int(temp_list[i]))
target_val = int(temp_list[64])
#output_val = calc_output(calc_output(x_list,percept_dic[target_val])) #target value 값과 같은 perceptron 에 대한 output 계산
for i in range(10): #0~9까지의 perceptron에 대하여
if i == target_val:
output_val = calc_output(x_list,percept_dic[i])
change_w_list(percept_dic[i],x_list,learning_rate,1,output_val) #해당 perceptron만 1로 학습
else:
output_val = calc_output(x_list,percept_dic[i])
change_w_list(percept_dic[i], x_list, learning_rate, 0, output_val) #나머지 perceptron은 0으로 학습
for i in range(10):
print(percept_dic[i])
test_data = open('optdigits.tes','r')
#test_data = open('b.txt','r')
correct_num = 0
wrong_num = 0
result_dic = {0 : 0 , 1 : 0, 2 : 0, 3 : 0, 4 : 0, 5: 0, 6 : 0, 7 : 0, 8 : 0, 9 : 0}
for line in test_data:
x_list = [1]
temp_list = line.split(',')
for i in range(64):
x_list.append(float(temp_list[i]))
for i in range(10):
#result_dic[i] = 1 - calc_output(x_list,percept_dic[i])
result_dic[i] = calc_output2(x_list, percept_dic[i])
print(result_dic)
#answer = min(result_dic, key=lambda key: result_dic[key])
answer = max(result_dic, key=lambda key: result_dic[key])
#print('답 : ' + str(answer))
true_num = int(temp_list[64])
if answer == true_num:
#print('correct')
correct_num = correct_num+1
else:
#print('wrong')
#print(result_dic)
#print(answer)
#print(true_num)
wrong_num = wrong_num+1
all_num = correct_num + wrong_num
success_percent = correct_num/all_num * 100
fail_percent = wrong_num/all_num * 100
print('Success Percent :' + str(success_percent))
print('Failure Percent :' + str(fail_percent))
#print(w_list)
<file_sep>import numpy as np
#2*3 array 생성 data type은 int32
x = np.array([[1,2,3], [4,5,6]], np.int32)
print(type(x)) #<class 'numpy.ndarray'>
print(x.shape) #(2,3)
print(x.dtype) #dtype('int32')
print(x[1,2]) #6
y = x[:,1]
print(y) #[2 5]
y[0] = 9
print(y) #[9 5]
print(x) #[[1 9 3] [4 5 6]]
x = np.ndarray(shape=(2,2), dtype=float, order='C') #2*2 float 타입행렬 생성
print(x)
y = np.ndarray((2,), buffer=np.array([1,2,3]),offset=np.int_().itemsize,
dtype=int)
print(y)<file_sep>#TSLA 회사의 주식데이터(2010-2014)를 가져와서 화면에 그래프로 변화를 그려주는 프로그램입니다.
import matplotlib.pyplot as plt
import numpy as np
from numpy import genfromtxt
#CSV 파일 열기
my_data = genfromtxt('TSLA_stockprice2.csv', delimiter=',' ,names=True)
fname = open('TSLA_stockprice2.csv','r')
#0번째에서 6번째 칼럼을 대상으로 각각의 그래프 생성
plt.plotfile(fname, (0, 1, 2, 3, 4, 5, 6))
#Open,High,Low,Close 각각의 최대값을 받아옴
max_open = np.max(my_data['Open'])
max_high = np.max(my_data['High'])
max_low = np.max(my_data['Low'])
max_close = np.max(my_data['Close'])
#분석결과를 콘솔창에 출력
print('***** TSLA_stockprice 분석 결과값 *****')
print('open의 최대값 : '+str(max_open))
print('high의 최대값 : '+str(max_high))
print('low의 최대값 : '+str(max_low))
print('close의 최대값 : '+str(max_close))
#최종적인 결과를 화면에 출력하기
plt.suptitle('TSLA_stockprice',fontsize = 20) #그래프 제목설정
plt.show()
<file_sep>file = open("input.txt",'r')
file2 = open("data_set.txt",'w')
file3 = open("test_set.txt",'w')
file4 = open("validation_set.txt",'w')
for i in range(5000):
file2.write(file.readline())
for i in range(500):
file4.write(file.readline())
for i in range(2624):
file3.write(file.readline())
<file_sep>import numpy as np
x = np.float32(1.0)
print(x)
y = np.int_([1,2,4])
print(y)
z = np.arange(3, dtype=np.uint8)
print(z)
temp = np.array([1,2,3], dtype = 'f')
print(temp)
temp = z.astype(float) #uint8 datatype을 float로 변경
print(temp)
temp = np.int8(z)
print(temp)
temp = z.dtype
print(temp)
d = np.dtype(int)
print(d)
temp = np.issubdtype(d, int) #d가 int타입이면 true 아니면 false를 반환
print(temp) # true
temp = np.issubdtype(d, float) #d가 float타입이면 true 아니면 false를 반환
print(temp) #false
<file_sep>import numpy as np
import copy
from collections import OrderedDict
class Node:
data = ''
def __init__(self):
self.child_dic = {}
self.attr_list = []
def print_child_dic(self): #child_dic를 만들고, attr_list도 만든다
print(self.child_dic)
def set_child_dic(self,attr_list):
for temp in attr_list:
self.child_dic[temp] = Node()
self.attr_list.append(temp)
def get_child_dic(self):
return self.child_dic
def print_nodes(self):
if self.data == 'empty':
print('empty')
return
print(self.data)
if self.data is 'e' or self.data is 'p': #terminal node이면 return
return
for temp in self.attr_list:
print(str(temp) + '일때')
self.child_dic[temp].print_nodes()
def calc_antrophy(list):
e_num = 0
p_num = 0
for temp in list:
if temp['classes'] == 'e':
e_num = e_num+1
elif temp['classes'] == 'p':
p_num = p_num+1
all_num = e_num + p_num
result = (-1) * e_num/all_num * np.log2(e_num/all_num) + (-1) * p_num/all_num * np.log2(p_num/all_num)
return result
def calc_antrophy2(list):
e_num = list[0]
p_num = list[1]
all_num = e_num + p_num
if e_num == 0 and p_num == 0:
return 0
elif e_num == 0 and p_num != 0:
result = (-1) * p_num / all_num * np.log2(p_num / all_num)
return result
elif p_num == 0 and e_num != 0:
result = (-1) * e_num / all_num * np.log2(e_num / all_num)
return result
result = (-1) * e_num / all_num * np.log2(e_num / all_num) + (-1) * p_num / all_num * np.log2(p_num / all_num)
return result
def calc_gain(total_entrophy, total_num, temp, dic_name, ent_list, attr_array):
result = total_entrophy
for i in dic_name[temp]:
result -= (attr_array[temp][i][0] + attr_array[temp][i][1])/total_num * ent_list[temp][i]
return result
def all_same(items): #list안에 값들이 서로 같은지 검사하는 함수
return all(x == items[0] for x in items)
def check_end(training_dic_list, attr_name_list): #끝인경우는 true를 반환 끝이 아닌경우는 false
#attrbute_name_list에 아무것도 없는 경우 or e,p값이 섞여있지 않은경우
if not attr_name_list:
print("List is empty!")
return True
#e,p 값 섞여있는지 판단
result_list = []
for temp in training_dic_list:
result_list.append(temp['classes'])
if all_same(result_list) is True:
return True
return False
def make_decision_tree(root_node,training_dic_list,attr_name_list,dic_name):
if not training_dic_list: #training_dic_list에 아무값도 없는경우(특정값을 가지는 데이터가 없는경우
print('empty node')
root_node.data = 'empty'
return
if check_end(training_dic_list,attr_name_list) is True: #종료조건
#terminal node를 등록
temp_node = make_terminal_node(training_dic_list,attr_name_list)
root_node.data = temp_node.data
return
total_num = len(training_dic_list)
print('total_num : ' + str(total_num))
D = calc_antrophy(training_dic_list)
print('D : '+str(D))
# 2) 22개의 attr 각각에 대한 Gain값 계산
attr_list = {}
attr_temp1 = {}
attr_ent = {}
attr_temp2 = {}
gain_list = {}
attr_temp3 = {}
for attr_name in attr_name_list[1:]:
for j in dic_name[attr_name]:
attr_temp1[j] = [0, 0]
attr_temp2[j] = 0
attr_list[attr_name] = attr_temp1
attr_ent[attr_name] = attr_temp2
gain_list[attr_name] = 0
attr_temp1 = {}
attr_temp2 = {}
# e,p 구분
for line in training_dic_list:
for temp in attr_name_list[1:]:
if line['classes'] == 'e':
attr_list[temp][line[temp]][0] += 1
elif line['classes'] == 'p':
attr_list[temp][line[temp]][1] += 1
# entrophy 계산
for temp in attr_name_list[1:]:
for temp2 in dic_name[temp]:
attr_ent[temp][temp2] = calc_antrophy2(attr_list[temp][temp2])
# gain 값 구하기
for temp in attr_name_list[1:]:
gain_list[temp] = calc_gain(D, total_num, temp, dic_name, attr_ent, attr_list)
# gain이 최대인 칼럼찾기
max_attr_name = max(gain_list, key=gain_list.get)
print('max_attr_name')
print(max_attr_name)
# 최대인 칼럼으로 노드생성 => attribute 종류만큼 child node 생성
#root_node = Node(max_attr_name,dic_name[max_attr_name])
root_node.data = max_attr_name
root_node.set_child_dic(dic_name[max_attr_name])
print('child_dic')
root_node.print_child_dic()
new_attribute_name_list = make_new_attribute_name_list(attr_name_list, max_attr_name)
#training data set 가공
for temp in dic_name[max_attr_name]:
print(temp)
new_training_data_list = make_new_training_data_list(training_dic_list,max_attr_name,temp)
make_decision_tree(root_node.child_dic[temp],new_training_data_list,new_attribute_name_list,dic_name)
return root_node
def make_terminal_node(training_dic_list,attr_name_list):
#결과값 모두 저장
result_list = []
for temp in training_dic_list:
result_list.append(temp['classes'])
print('attr_name_list')
print(attr_name_list)
if not attr_name_list: #attr_name_list가 비어있는경우
print('empty_attr_list!!!!!!!!!!!')
if all_same(result_list) is True: # attr_name_list에 아무것도 없으면서(끝까지 가지를 뻗은경우) 값이 모두 동일한 경우
terminal_node = Node(result_list[0])
print("List is empty and all value is same!")
elif all_same(result_list) is False: # attr_name_list에 아무것도 없으면서(끝까지 가지를 뻗은경우) 값이 섞여있는 경우
print("terminal에서 다수결로 결정")
# result_set에서 더 많은 값으로 선택
if result_list.count('e') >= result_list.count('p'):
terminal_node = Node('e')
else:
terminal_node = Node('p')
else: #attr_name_list에 값이 있지만 결과값이 모두 동일한 경우
print('NOT empty')
terminal_node = Node()
terminal_node.data = result_list[0]
print("List is NOT empty BUT all value is same!")
return terminal_node
def make_new_training_data_list(training_data_list, attr_name, value): #value와 일치하는 값을 가지는 데이터셋만 찾아서 반환
new_training_data_list = []
for temp in training_data_list:
if temp[attr_name] == value:
new_training_data_list.append(temp)
return new_training_data_list
def make_new_attribute_name_list(attr_name_list,attr_name):
for i in range(len(attr_name_list)):
if attr_name_list[i] == attr_name:
attr_name_list.pop(i)
return attr_name_list
def func_test_data(root_node, test_dic_list):
all_num = len(test_dic_list)
correct_num = 0
wrong_num = 0
for test_dic in test_dic_list: #총 데이터의 수만큼 반복
temp_node = root_node
print(temp_node.data)
while is_terminal(temp_node.data) is False: #terminal이 될때 까지 반복
print('node_data')
node_data = test_dic[temp_node.data]
print(node_data)
temp_node = temp_node.child_dic[node_data]
print('result : ' + temp_node.data)
print('real value : ' + test_dic['classes'])
if (temp_node.data == test_dic['classes']):
correct_num+=1
else:
wrong_num+=1
suc_percent = (correct_num/all_num) * 100
fail_percent = (wrong_num/all_num) * 100
print(correct_num)
print(wrong_num)
print('success percentage : ' + str(suc_percent))
print('fail percentage : ' + str(fail_percent))
def is_terminal(data):
if data == 'e' or data == 'p' or data == 'empty':
return True
return False
#################################################################
#mushroom.names file 읽어서, dictionary에 저장 eg. { 'classes' : {e,p}, ....}
file_msuhroom_names = open("mushroom.names",'r')
dic_name = OrderedDict()
for line in file_msuhroom_names:
temp = line.split(' ')
attr_values = []
for item in temp[1:]:
attr_values.append(item.strip('\n'))
dic_name[temp[0]] = attr_values
print('dic_name')
print(dic_name)
#attr name을 저장
items = list(dic_name.items())
attr_name_list = []
for temp in items:
attr_name_list.append(temp[0])
print('attr_name_list')
print(attr_name_list)
#training data를 파일로 부터 읽어서 dictionary 형태로 변환
training_data = open('training_set.txt','r')
#training_data = open('a.txt','r')
training_dic_list = []
for line in training_data:
temp1 = line.split(',')
i = 0
training_dic = {}
for temp2 in attr_name_list:
training_dic[temp2] = temp1[i].strip('\n')
i+=1
training_dic_list.append(training_dic)
print('********************************************')
new_attr_name_list = copy.deepcopy(attr_name_list)
root_node = Node()
root_node = make_decision_tree(root_node,training_dic_list,new_attr_name_list, dic_name)
print('*********************************************')
root_node.print_nodes()
#test_data = open('test_set.txt','r')
#test_data = open('validation_set.txt','r')
#test_data = open('b.txt','r')
test_data = open('input.txt','r')
test_dic_list = []
for line in test_data:
temp1 = line.split(',')
i = 0
test_dic = {}
for temp2 in attr_name_list:
test_dic[temp2] = temp1[i].strip('\n')
i+=1
test_dic_list.append(test_dic)
func_test_data(root_node,test_dic_list)
<file_sep>from scipy.integrate import quad
def integrand(x,a,b):
return a*x**2 + b
a = 2
b = 1
I = quad(integrand, 0, 1, args=(a,b)) #ax제곱 + b 함수를 0~1 구간으로 정적분
print(I)
<file_sep>from nltk.stem.snowball import SnowballStemmer
import re
import math
import copy
import os
def make_stopword_list(f_name):
f = open(f_name)
for stopword in f:
stopword_list = stopword.split(' ')
return stopword_list
#delete stopword(s) in word list
def delete_stopword(word_list, stopword_list):
for i in range(len(stopword_list)):
if stopword_list[i] in word_list:
word_list.remove(stopword_list[i])
return
def UseStemmer(word_list,stemmer_obj):
word_cnt = len(word_list)-2
for i in range(1,word_cnt+1):
word_list[i] = stemmer_obj.stem(word_list[i])
return
def delete_word(complete_dic, word_dic):
acq_word_list = word_dic['acq']
etc_word_list = word_dic['etc']
delete_acq_word = []
delete_etc_word = []
acq_word_cnt_dic = complete_dic['acq']
etc_word_cnt_dic = complete_dic['etc']
#delete from cnt_dic
for word in acq_word_list:
if acq_word_cnt_dic[word] < 5:
delete_acq_word.append(word)
acq_word_cnt_dic.pop(word)
for word in etc_word_list:
if etc_word_cnt_dic[word] < 5:
delete_etc_word.append(word)
etc_word_cnt_dic.pop(word)
#delete form word_list
for word in acq_word_list:
if word in delete_acq_word:
acq_word_list.pop(word)
for word in etc_word_list:
if word in delete_etc_word:
etc_word_list.pop(word)
return
def make_word_dic(file_name, stemmer, stopword_list):
word_dic = {}
f = open(file_name)
for line in f:
line_list = re.split('\t| ', line)
delete_stopword(line_list, stopword_list)
UseStemmer(line_list, stemmer)
if line_list[0] == 'acq':
input_class = line_list[0]
else:
input_class = 'etc'
word_cnt = len(line_list) - 2
# Check input_class is in input_dic
if input_class not in word_dic:
word_dic[input_class] = []
word_list = word_dic[input_class]
for i in range(1, word_cnt + 1):
input_word = line_list[i]
if input_word in word_list:
continue
else:
word_list.append(input_word)
return word_dic
def combine_two_word_list(word_list1, word_list2):
new_word_list = copy.deepcopy(word_list1)
for word2 in word_list2:
if word2 not in new_word_list:
new_word_list.append(word2)
return new_word_list
def make_word_list(complete_word_dic):
acq_word_list = complete_word_dic['acq']
etc_word_list = complete_word_dic['etc']
new_word_list = combine_two_word_list(acq_word_list,etc_word_list)
return new_word_list
def make_output_dictionary(file_name, stemmer, stopword_list ):
output_dic = {}
f = open(file_name)
num_of_doc = 0
# make dictionary => {'acq' : { 'word' : 0, ... } , 'etc' : { 'word' : 0, ... } }
for line in f:
num_of_doc += 1
# line list contains => first element : class , last element : newline
line_list = re.split('\t| ', line)
delete_stopword(line_list, stopword_list)
UseStemmer(line_list, stemmer)
if line_list[0] == 'acq':
input_class = line_list[0]
else:
input_class = 'etc'
word_cnt = len(line_list) - 2
# Check input_class is in input_dic
if input_class not in output_dic:
output_dic[input_class] = {}
word_list = output_dic[input_class]
# make word in class list or increase the count
for i in range(1, word_cnt + 1):
input_word = line_list[i]
if input_word in word_list:
word_list[input_word] += 1
else:
word_list[input_word] = 1
return output_dic,num_of_doc
def calc_all_value(dic,list):
all_num = 0
for temp in list:
all_num += dic[temp]
return all_num
def calc_tf_idf(word_cnt,all_acq_word_num,num_of_doc,doc_cnt):
term_freq = word_cnt/all_acq_word_num
inv_doc_freq = math.log(num_of_doc/doc_cnt)
result = term_freq * inv_doc_freq
return result
def make_doc_cnt_dic(file_name, stemmer, stopword_list,complete_word_list):
word_doc_cnt = {}
for word in complete_word_list:
word_doc_cnt[word] = 0
f = open(file_name)
for line in f:
line_list = re.split('\t| ', line)
delete_stopword(line_list, stopword_list)
UseStemmer(line_list, stemmer)
for word in complete_word_list:
if word in line_list:
word_doc_cnt[word] += 1
return word_doc_cnt
def make_tf_idf_dic(complete_dic,complete_word_dic,all_word_list,num_of_doc,doc_cnt_dic):
output_dic = {}
acq_cnt_dic = complete_dic['acq']
acq_word_list = complete_word_dic['acq']
all_acq_word_num = calc_all_value(acq_cnt_dic,acq_word_list)
for word in all_word_list:
if word in acq_word_list:
output_dic[word] = calc_tf_idf(acq_cnt_dic[word],all_acq_word_num,num_of_doc,doc_cnt_dic[word])
else:
output_dic[word] = 0
return output_dic
##################functions for Adaboost###################
def make_expected_val_list(file_name):
expected_val_list = []
f = open(file_name)
for line in f:
line_list = re.split('\t| ', line)
if line_list[0] == 'acq':
expected_val_list.append(1)
else:
expected_val_list.append(-1)
return expected_val_list
def make_output_list(input_file_name):
input_file = open(input_file_name)
output_list = []
for output_val in input_file:
if float(output_val) > 0 :
output_list.append(1)
else:
output_list.append(-1)
return output_list
def init_weight_list(weight_list,num_of_data):
for i in range(num_of_data):
weight_list.append(1/num_of_data)
return
def calc_sum_of_weight(expected_val_list, weight_list, output_list, num_of_data):
sum = 0
for i in range(num_of_data):
sum += weight_list[i] * math.exp( (-1) * expected_val_list[i] * output_list[i])
return sum
def updata_weights(weight_list,expected_val_list,output_list,alpha_val,num_of_data):
for i in range(num_of_data):
weight_list[i] = weight_list[i] * math.exp((-1)*expected_val_list[i]*output_list[i]*alpha_val)
return
def make_new_weight_list(input_file_name, expected_val_list, num_of_data):
weight_list = []
init_weight_list(weight_list,num_of_data)
output_list = make_output_list(input_file_name)
sum_of_weight = calc_sum_of_weight(expected_val_list, weight_list,output_list,num_of_data)
alpha_val = math.log((1-sum_of_weight)/sum_of_weight)/2
updata_weights(weight_list,expected_val_list,output_list,alpha_val,num_of_data)
return weight_list
def make_new_svm_input(input_file_name,stopword_list,stemmer,output_file_name,tf_idf_dic,all_word_list,weight_list):
input_file = open(input_file_name)
output_file = open(output_file_name,'w')
i = 0
for line in input_file:
# line list contains => first element : class , last element : newline
output_str = ''
line_list = re.split('\t| ', line)
delete_stopword(line_list, stopword_list)
UseStemmer(line_list, stemmer)
word_cnt = len(line_list) - 2
#extract words from line_list AND make sorted list ascending order
temp_list = line_list[1:word_cnt+1]
sorted_list = []
for word in all_word_list:
if word in temp_list:
sorted_list.append(word)
#make output stirng
if line_list[0] == 'acq':
output_str += '1'
else:
output_str += '-1'
for word in sorted_list:
index = all_word_list.index(word)+1
if tf_idf_dic[word] == 0:
continue
output_str += ' '+str(index) + ':' + str(tf_idf_dic[word]*weight_list[i])
output_str += '\n'
output_file.write(output_str)
i+=1
return
def make_svm_input(input_file_name,stopword_list,stemmer,output_file_name,tf_idf_dic,all_word_list):
input_file = open(input_file_name)
output_file = open(output_file_name,'w')
for line in input_file:
# line list contains => first element : class , last element : newline
output_str = ''
line_list = re.split('\t| ', line)
delete_stopword(line_list, stopword_list)
UseStemmer(line_list, stemmer)
word_cnt = len(line_list) - 2
#extract words from line_list AND make sorted list ascending order
temp_list = line_list[1:word_cnt+1]
sorted_list = []
for word in all_word_list:
if word in temp_list:
sorted_list.append(word)
#make output stirng
if line_list[0] == 'acq':
output_str += '1'
else:
output_str += '-1'
for word in sorted_list:
index = all_word_list.index(word)+1
if tf_idf_dic[word] == 0:
continue
output_str += ' '+str(index) + ':' + str(tf_idf_dic[word])
output_str += '\n'
output_file.write(output_str)
return
def test_exec(output_f_name1,output_f_name2,expected_list,alpha1,alpha2,len):
output_list1 = make_output_list(output_f_name1)
output_list2 = make_output_list(output_f_name2)
true_num = 0
for i in range(len):
new_output = output_list1[i] * alpha1 + output_list2[i] * alpha2
if (new_output > 0):
new_output = 1
else:
new_output = -1
if new_output == expected_list[i]:
true_num += 1
print('success rate : ' + (str)(true_num/len))
##################################MAIN###################################
alpha1 = 0
alpha2 = 0
#make stemmer object and stopword list
stemmer = SnowballStemmer("english", ignore_stopwords=False)
stop_word_f_name = 'stopword.txt'
stopword_list = make_stopword_list(stop_word_f_name)
#make word list from train set, tf*idf dictionay => {word1 : 0.xxxx, word2 : 0.xxxx, word3 : 0.xxxx, ....}
train_input_f_name = 'train.txt'
complete_output_dic,num_of_doc = make_output_dictionary(train_input_f_name,stemmer,stopword_list)
complete_word_dic = make_word_dic(train_input_f_name,stemmer,stopword_list)
complete_word_list = make_word_list(complete_word_dic)
doc_cnt_dic = make_doc_cnt_dic(train_input_f_name,stemmer,stopword_list,complete_word_list)
tf_idf_dic = make_tf_idf_dic(complete_output_dic,complete_word_dic,complete_word_list,num_of_doc,doc_cnt_dic)
#make expected value list
expected_val_list = make_expected_val_list(train_input_f_name)
#make output files
first_output_name = 'input1.txt'
second_output_name = 'input2.txt'
output_f1_name = 'output1.txt'
output_f2_name = 'output2.txt'
weight_list = []
init_weight_list(weight_list,len(expected_val_list))
make_new_svm_input(train_input_f_name,stopword_list,stemmer,first_output_name,tf_idf_dic,complete_word_list,weight_list)
print('################### model1 생성 ######################')
os.system("./svm_learn input1.txt model1.txt")
os.system("./svm_classify input1.txt model1.txt "+ output_f1_name)
new_weight_list = make_new_weight_list(output_f1_name,expected_val_list,len(expected_val_list))
make_new_svm_input(train_input_f_name,stopword_list,stemmer,second_output_name,tf_idf_dic,complete_word_list,new_weight_list)
print('################### model2 생성 ######################')
os.system("./svm_learn input2.txt model2.txt")
os.system("./svm_classify input1.txt model2.txt "+ output_f2_name)
#expected_val_list2 = make_expected_val_list('test.txt')
'''
test_input_f_name = 'test.txt'
test_output_f_name = 'new_test.txt'
output_f_name = 'output.txt'
make_new_svm_input(test_input_f_name,stopword_list,stemmer,test_output_f_name,tf_idf_dic,complete_word_list,new_weight_list)
make_new_svm_input(test_input_f_name,stopword_list,stemmer,test_output_f_name,tf_idf_dic,complete_word_list,new_weight_list)
'''
<file_sep>#polynomials
import numpy as np
from numpy import poly1d
p = poly1d([3,4,5]) #3x+4x+5
print(p)
print(p*p) #9x + 24x + 46x + 40x + 25
#Vectorizing functions
def addsubtract(a,b):
if a > b:
return a-b
else:
return a+b
vec_addsubtract = np.vectorize(addsubtract)
print(vec_addsubtract([0,3,6,9], [1,3,5,7]))
#Type handling
print(np.cast['f'](np.pi)) #3.1415927410125732
#Other useful functions
x = np.r_[-2:3]
print(x)
print(np.select([x > 3, x >= 0], [0,x+2]))
<file_sep>import numpy as np
a = np.array([1.0,2.0,3.0])
b = np.array([2.0,2.0,2.0])
print(a*b)
a = np.array([1.0,2.0,3.0])
b= 2.0
print(a*b)
#General Boradcasting Rules
x = np.arange(4)
xx = x.reshape(4,1)
y = np.ones(5) #1 다섯개로 이루어진 1D 배열
print(y)
z = np.ones((3,4))
print(xx + y) # 4X5행렬 생성
a = np.array([0.0,10.0,20.0,30.0])
b = np.array([1.0,2.0,3.0])
temp = a[:,np.newaxis] + b
print(temp.shape) #4X3 행렬생성
print(temp)
<file_sep>import random
import math
def sigmoid(x):
return 1 / (1 + math.exp(-x))
def calc_output(x_list,w_list):
result = 0
for i in range(len(x_list)):
result = result + x_list[i]*w_list[i]
result = sigmoid(result)
return result
def calc_real_output(x_list,w_list):
result = 0
for i in range(len(x_list)):
result = result + x_list[i] * w_list[i]
return result
def calc_output_delta(target, output_val):
result = output_val * (1-output_val) * (target - output_val)
return result
def calc_hidden_delta(output_percept_dic,output_delta_dic,hidden_node_num, output_val):
sum = 0
for i in range(10):
sum += output_delta_dic[i]*output_percept_dic[i][hidden_node_num]
result = output_val * (1-output_val)*sum
return result
#output layer에서 output node 한개에 대한 w_list 수정
def change_w_list_output(w_list,x_list,learning_rate,target,output):
for i in range(len(w_list)):
w_list[i] = w_list[i] + learning_rate*calc_output_delta(target,output)*x_list[i]
return
#hidden layer에서 hidden node 한개에 대한 w_list 수정
def change_w_list_hidden(hidden_w_list,learning_rate,hidden_delta_val,x_list):
for i in range(len(hidden_w_list)):
hidden_w_list[i] = hidden_w_list[i] + learning_rate*hidden_delta_val*x_list[i]
return
learning_rate = 0.05
#output layer, hidden layer 선언
output_percept_dic = {0 : [], 1 : [], 2 : [], 3 : [], 4 : [], 5 : [], 6 : [], 7 : [], 8 : [], 9 : []}
hidden_percept_dic = {0 : [], 1 : [], 2 : [], 3 : [], 4 : [], 5 : [], 6 : [], 7 : [], 8 : [], 9 : []}
output_delta_dic = {0 : 0, 1 : 0, 2 : 0, 3 : 0, 4 : 0, 5 : 0, 6 : 0, 7 : 0, 8 : 0, 9 : 0}
hidden_delta_dic = {0 : 0, 1 : 0, 2 : 0, 3 : 0, 4 : 0, 5 : 0, 6 : 0, 7 : 0, 8 : 0, 9 : 0}
for i in range(10):
for j in range(10):
output_percept_dic[j].append(random.uniform(-0.125,0.125))
for i in range(10):
for j in range(65):
hidden_percept_dic[i].append(random.uniform(-0.125,0.125))
training_data = open('optdigits.tra','r')
#training_data = open('a.txt','r')
for i in range(50):
training_data = open('optdigits.tra', 'r')
for line in training_data:
output_layer_output = []
hidden_layer_output = []
x_list = [1]
temp_list = line.split(',')
for i in range(64):
x_list.append(int(temp_list[i]))
target_val = int(temp_list[64])
#Forward Propagation
for i in range(10):
hidden_layer_output.append(calc_output(x_list,hidden_percept_dic[i]))
for i in range(10):
output_layer_output.append(calc_output(hidden_layer_output,output_percept_dic[i]))
#Backward Propagation
#output layer w값수정
for i in range(10):
if target_val == i:
change_w_list_output(output_percept_dic[i],hidden_layer_output,learning_rate,1,output_layer_output[i])
# output_delta 값 구하기
output_delta_dic[i] = calc_output_delta(1, output_layer_output[i])
else:
change_w_list_output(output_percept_dic[i], hidden_layer_output, learning_rate, 0, output_layer_output[i])
#output_delta 값 구하기
output_delta_dic[i] = calc_output_delta(0, output_layer_output[i])
#hidden layer의 delta값 구하기
for i in range(10):
hidden_delta_dic[i] = calc_hidden_delta(output_percept_dic,output_delta_dic,i,hidden_layer_output[i])
#hidden layer w값수정
for i in range(10):
change_w_list_hidden(hidden_percept_dic[i],learning_rate,hidden_delta_dic[i],x_list)
print('output layer:')
for i in range(10):
print(i)
print(output_percept_dic[i])
print('hidden layer:')
for i in range(10):
print(i)
print(hidden_percept_dic[i])
test_data = open('optdigits.tes','r')
#test_data = open('b.txt','r')
correct_num = 0
wrong_num = 0
result_dic = {0 : 0 , 1 : 0, 2 : 0, 3 : 0, 4 : 0, 5: 0, 6 : 0, 7 : 0, 8 : 0, 9 : 0}
print('####### test #######')
for line in test_data:
hidden_layer_output = []
x_list = [1]
temp_list = line.split(',')
for i in range(64):
x_list.append(int(temp_list[i]))
#hidden_layer_output 생성
for i in range(10):
hidden_layer_output.append(calc_output(x_list,hidden_percept_dic[i]))
#output_layer_output 생성 -> result_dic에 저장
for i in range(10):
result_dic[i] = calc_output(hidden_layer_output,output_percept_dic[i])
#answer = min(result_dic, key=lambda key: result_dic[key])
answer = max(result_dic, key=lambda key: result_dic[key]) #값중에서 최대값을 구함
#print(answer)
#print('답 : ' + str(answer))
true_num = int(temp_list[64])
if answer == true_num:
print(true_num)
print(result_dic)
correct_num = correct_num+1
else:
#print('wrong')
#print(result_dic)
wrong_num = wrong_num+1
all_num = correct_num + wrong_num
success_percent = correct_num/all_num * 100
fail_percent = wrong_num/all_num * 100
print('Success Percent :' + str(success_percent))
print('Failure Percent :' + str(fail_percent))
#print(w_list)
| 487faa389941a3d542bb94e3cf61aebbf5d78c35 | [
"Markdown",
"Python"
] | 25 | Python | JaeguKim/AI_2017_spring_semester | 9dbeeb724783eec5340d6aca45f82887628ac64f | b8c7749abcfa256f040563d854cb20f71de88b7d | |
refs/heads/master | <file_sep># worker-union
Every Node.JS developer is aware of the single-threaded nature of this platform
where everything except your Javascript code runs in parallel..
But what if I tell you that it has become possible to run JS in parallel as well?
The worker_threads module has been added to node.js v10.5.0,
which allows javascript to be executed in separate threads within a single Node.JS process.
Yes, no spawn, exec, fork, cluster and other parodies on multithreading!
Worker-union allows you to take advantage of worker_threads,
while eliminating the need to manually manage messaging,
the number of threads, load balancing between them, and so on.
Just write logic, the rest will be done by promise-powered worker-union 🤓
Let’s jump into it?
## Requirements
Since module 'worker_threads' was added only in __Node.JS v10.5.0__,
you cannot use 'worker-union' in earlier versions.
Also, when you start your application, you will need to use `--experimental-worker` flag
(ex. `node --experimental-worker index.js`)
## Install
```bash
npm i -S worker-union
```
## Workerify
You can save yourself from the boilerplate at all, using the `workerify`!
Just pass on your function and get an asynchronous wrapper.
The code of the function itself will be executed in the Worker Pool.
```javascript
const workerify = require('worker-union/workerify');
const parseJSON = value => JSON.parse(value);
const asyncParseJSON = workerify(parseJSON);
asyncParseJSON('{"foo":"bar"}').then(result => result.foo); // -> bar
```
It is important to know that your function must be serializable.
You can easily verify this using `func.toString()` method. If you want to workerify the native Node.JS function,
for example _JSON.parse()_ (when you call `func.toString()` you see `[native code]`), then wrap it as in the example above.
By default, _worker-union_ will allocate for your function the number of threads
equal to the number of cores of your processor. If you want to tune the WorkerPool settings by yourself,
pass them as second argument to `workerify` function:
```javascript
const asyncFn = workerify(fn, {
count: 4,
});
```
## Basic usage of WorkerPool
In this example, work with the worker on the __"request-response"__ principle will be demonstrated.
__Project structure:__
```
. your-project-directory
└── node_modules
└── index.js
└── worker.js
```
__index.js:__
```javascript
const path = require('path');
const WorkerPool = require('worker-union');
const pool = new WorkerPool({
path: path.resolve('worker.js'), // absolute path to worker source code
count: 1,
});
pool.start();
pool.send('ping').then(response => console.log(response));
```
__worker.js__
```javascript
const Worker = require('worker-union/worker');
new Worker((message) => {
console.log('Received message:', message);
return 'pong';
});
```
__Result:__
```bash
node --experimental-worker index.js
# -> pong
```
## Another WorkerPool example
And in this example it will be shown that the worker can send messages to the main thread __without requests__.
__Project structure:__
```
. your-project-directory
└── node_modules
└── index.js
└── worker.js
```
__index.js:__
```javascript
const path = require('path');
const WorkerPool = require('worker-union');
const pool = new WorkerPool({
path: path.resolve('worker.js'),
count: 1,
eventHandler: message => console.log(`Recieved message from worker: ${message}`),
});
pool.start();
// we doesn't send any data here
```
__worker.js__
```javascript
const Worker = require('worker-union/worker');
const worker = new Worker();
setInterval(() => worker.emit('Hello!'), 1000); // send 'Hello' to main thread every second
```
__Result:__
```bash
node --experimental-worker index.js
# -> Received message from worker: Hello!
# -> Received message from worker: Hello!
# -> Received message from worker: Hello!
# -> Received message from worker: Hello!
```
## Advanced features
A description of all the features of the library, such as automatic thread allolocation, shared-memory state etc., will be ready soon. Stay tuned!
## License
MIT.
<file_sep>const workerThreads = require('worker_threads');
if (!workerThreads.isMainThread) {
const {
workerData: {
bufferAccessMap,
stateDataView,
initialData,
},
parentPort,
threadId: workerId,
} = workerThreads;
module.exports = class Worker {
constructor(handler) {
this.initialData = initialData;
this.id = workerId;
this.state = new Proxy({}, {
get: (target, key) => Boolean(stateDataView.getUint8(bufferAccessMap.get(key))),
set: (target, key, value) => stateDataView.setUint8(bufferAccessMap.get(key), value ? 1 : 0),
});
if (handler !== undefined) {
this.setMessageHandler(handler);
}
}
static getWorkerId() {
return workerId;
}
emit(data) {
parentPort.postMessage({
isEvent: true,
workerId,
data,
});
}
setMessageHandler(fn) {
parentPort.on('message', async ({ messageId, data }) => {
const resolve = response => parentPort.postMessage({
failed: false,
data: response,
messageId,
workerId,
});
const reject = error => parentPort.postMessage({
failed: true,
data: error,
messageId,
workerId,
});
try {
const result = await fn(data);
resolve(result);
} catch ({ message, stack }) {
// errors are not clonable objects, so we do this
reject({ message, stack });
}
});
}
};
} else {
throw new Error('This module should only be used in your worker file. You cannot use it in the main thread.');
}
<file_sep>const path = require('path');
const WorkerPool = require('../WorkerPool');
const workerPath = path.join(__dirname, 'worker.js');
/* eslint-disable no-param-reassign */
module.exports = (func, options = {}) => {
delete options.initialData;
delete options.path;
const pool = new WorkerPool({
initialData: func.toString(),
path: workerPath,
...options,
});
pool.start();
return (...args) => pool.send(args);
};
<file_sep>module.exports = require('./src/workerify');
<file_sep>const os = require('os');
const { Worker } = require('worker_threads');
const generateRandomString = require('./utils/generateRandomString');
const cpuCoresCount = os.cpus().length;
module.exports = class WorkerPool {
constructor({
path,
state = {},
autoAllocation = false,
minCount = 2,
maxCount = cpuCoresCount,
count = cpuCoresCount,
messagesLimit = 10,
eventHandler = () => {},
initialData,
}) {
this.workerFilePath = path;
this.initialData = initialData;
this.autoAllocation = autoAllocation;
this.messagesLimit = messagesLimit;
this.defaultWorkersCount = count;
this.minWorkersCount = minCount;
this.maxWorkersCount = autoAllocation ? maxCount : count;
this.workerMap = new Map();
this.messageMap = new Map(); // messageId -> { resolve, reject }
this.eventHandler = eventHandler;
this.initializeState(state);
}
start() {
const workerLoopLimit = this.autoAllocation ? this.minWorkersCount : this.defaultWorkersCount;
for (let i = 0; i < workerLoopLimit; i++) {
this.spawnWorker();
}
if (this.autoAllocation) setInterval(this.manageWorkersCount.bind(this), 1000);
}
reload() {
for (const [workerId] of this.workerMap) {
this.killWorker(workerId);
}
this.start();
}
initializeState(state) {
const stateBuffer = new SharedArrayBuffer(Object.keys(state).length * 4);
this.stateDataView = new DataView(stateBuffer);
this.bufferAccessMap = Object.keys(state).reduce((map, key, i) => map.set(key, i), new Map());
this.state = Object.keys(state).reduce((acc, key) => Object.defineProperty(acc, key, {
get: () => Boolean(this.stateDataView.getUint8(this.bufferAccessMap.get(key))),
set: value => this.stateDataView.setUint8(this.bufferAccessMap.get(key), value ? 1 : 0),
}), {});
}
get currentWorkersCount() {
return this.workerMap.size;
}
get overageMessageAmountPerWorker() {
let totalMessagesCount = 0;
for (const { messagesCount } of this.workerMap.values()) {
totalMessagesCount += messagesCount;
}
return totalMessagesCount / this.currentWorkersCount;
}
manageWorkersCount() {
const workers = this.currentWorkersCount;
const messages = this.overageMessageAmountPerWorker;
const min = this.minWorkersCount;
const max = this.maxWorkersCount;
if (workers === min) {
if (messages > this.messagesLimit) {
this.spawnWorker();
}
} else if (workers > min && workers < max) {
if (messages > this.messagesLimit) {
this.spawnWorker();
return;
}
this.killWorker();
} else if (workers === max) {
if (messages <= this.messagesLimit) {
this.killWorker();
}
}
}
spawnWorker() {
if (this.currentWorkersCount >= this.maxWorkersCount) {
console.warn('Maximum number of workers reached');
return;
}
const worker = new Worker(this.workerFilePath, {
workerData: {
bufferAccessMap: this.bufferAccessMap,
stateDataView: this.stateDataView,
initialData: this.initialData,
},
});
worker.on('message', this.handleMessage.bind(this));
this.workerMap.set(worker.threadId, {
isTerminating: false,
messagesCount: 0,
worker,
});
}
killWorker(workerId) {
if (this.currentWorkersCount === this.minWorkersCount) {
console.warn('Minimum number of workers reached');
return;
}
if (workerId !== undefined) {
this.workerMap.get(workerId).worker.terminate();
this.workerMap.delete(workerId);
return;
}
const targetWorkerId = this.getLeastLoadedWorkerId();
this.workerMap.get(targetWorkerId).isTerminating = true;
}
handleMessage({
isEvent = false,
workerId,
messageId,
data,
failed,
}) {
if (isEvent) {
this.eventHandler(data);
return;
}
const { resolve, reject } = this.messageMap.get(messageId);
if (failed) {
// errors are not clonable objects, so we do this
const e = new Error();
Object.assign(e, data);
reject(e);
} else {
resolve(data);
}
const workerData = this.workerMap.get(workerId);
workerData.messagesCount--;
if (workerData.isTerminating && workerData.messagesCount === 0) {
this.killWorker(workerId);
}
}
getLeastLoadedWorkerId() {
let leastCount = Infinity;
let chosenWorkerId;
for (const [workerId, { messagesCount, isTerminating }] of this.workerMap) {
if (isTerminating && messagesCount === 0) {
this.killWorker(workerId);
continue;
}
if (leastCount > messagesCount) {
leastCount = messagesCount;
chosenWorkerId = workerId;
}
}
return chosenWorkerId || this.workerMap.keys().next().value;
}
send(data, forcedWorkerId) {
const promiseMethods = {};
const promise = new Promise((resolve, reject) => {
promiseMethods.resolve = resolve;
promiseMethods.reject = reject;
});
const messageId = generateRandomString();
const workerId = forcedWorkerId || this.getLeastLoadedWorkerId();
this.messageMap.set(messageId, promiseMethods);
const workerData = this.workerMap.get(workerId);
workerData.worker.postMessage({ messageId, data });
workerData.messagesCount++;
return { workerId, promise };
}
async broadcast(data, sequentially = false) {
let promises = [];
for (const [workerId] of this.workerMap) {
const { promise } = this.send(data, workerId);
if (sequentially) await promise;
promises.push(promise);
}
return Promise.all(promises);
}
};
<file_sep>const Worker = require('../Worker');
const worker = new Worker();
// eslint-disable-next-line no-eval
const func = eval(worker.initialData);
worker.setMessageHandler(async (args, resolve, reject) => {
try {
resolve(await func(...args));
} catch (e) {
reject(e.message);
}
});
| 182966728383ef5661b9f15069f3abc52d5c7b8d | [
"Markdown",
"JavaScript"
] | 6 | Markdown | AlexTolmachev/node-worker-union | b30c290f3dd598ac2a13ffc80ee9bd30f5df2da2 | 9bfcf04fcab802d4f5425c353c2471d566cb7c89 | |
refs/heads/master | <repo_name>pelma24/AdventOfCode<file_sep>/2022/day5.py
from copy import deepcopy
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
import re
inputArrangement = [['N','T','B','S','Q','H','G','R'], ['J','Z','P','D','F','S','H'], ['V','H','Z'], ['H','G','F','J','Z','M'], ['R','S','M','L','D','C','Z','T'], ['J','Z','H','V','W','T','M'], ['Z','L','P','F','T'], ['S','W','V','Q'], ['C','N','D','T','M','L','H','W']]
def do1(splitInput):
arrangement = deepcopy(inputArrangement)
for line in splitInput:
match = re.match('move (?P<number>\d+) from (?P<start>\d) to (?P<end>\d)', line)
start = int(match.group('start')) - 1
number = int(match.group('number'))
end = int(match.group('end')) - 1
for _ in range(number):
arrangement[end].insert(0, arrangement[start].pop(0))
result = ''
for part in arrangement:
result = result + part[0]
return result
def do2(splitInput):
arrangement = deepcopy(inputArrangement)
for line in splitInput:
match = re.match('move (?P<number>\d+) from (?P<start>\d) to (?P<end>\d)', line)
start = int(match.group('start')) - 1
number = int(match.group('number'))
end = int(match.group('end')) - 1
movedParts = arrangement[start][0:number]
arrangement[start] = arrangement[start][number:]
arrangement[end] = movedParts + arrangement[end]
result = ''
for part in arrangement:
result = result + part[0]
return result
def do():
strInput = readInputFile(5)
splitInput = strInput.split('\n')
print(do1(splitInput))
print(do2(splitInput))
print('done')
do()<file_sep>/2020/day5.py
from HelperFunctions import inputsplit
def do1(puzzleInput):
seatIDs = getSeatIDs(puzzleInput)
return max(seatIDs)
def do2(puzzleInput):
seatIDs = getSeatIDs(puzzleInput)
seatIDs.sort()
seatBeforeMissingSeat = [x for x in range(len(seatIDs) - 1) if seatIDs[x + 1] - seatIDs[x] > 1]
return seatIDs[seatBeforeMissingSeat[0]] + 1
def getSeatIDs(puzzleInput):
seatIDs = []
for boardingPass in puzzleInput:
seatID = calculateSeatID(boardingPass)
seatIDs.append(seatID)
return seatIDs
def calculateSeatID(boardingPass):
seatID = 0
rowCalc = boardingPass[0:7]
columnCalc = boardingPass[7:]
row = calculatePosition(rowCalc, 127)
column = calculatePosition(columnCalc, 7)
seatID = row * 8 + column
return seatID
def calculatePosition(instructions, upper):
lower = 0
for instruction in instructions:
if instruction in ['F', 'L']:
upper = lower + int((upper - lower) / 2)
else:
lower = lower + int((upper - lower) / 2) + 1
return upper
def do():
with open ('Input/day5.txt') as f:
strInput = f.read()
splitInput = inputsplit(strInput, '\n')
print(do1(splitInput))
print(do2(splitInput))
do()<file_sep>/2020/day3.py
from HelperFunctions import inputsplit
def do1(puzzleInput):
return countTrees(puzzleInput, 1, 3)
def do2(puzzleInput, slopes):
trees = 1
for slope in slopes:
trees *= countTrees(puzzleInput, slope[0], slope[1])
return trees
def countTrees(puzzleInput, slopeRow, slopeColumn):
trees = 0
columnLength = len(puzzleInput[0])
column = 0
for row in range(slopeRow, len(puzzleInput), slopeRow):
column = (column + slopeColumn) % columnLength
if puzzleInput[row][column] == '#':
trees += 1
return trees
def do():
with open ('Input/day3.txt') as f:
strInput = f.read()
splitInput = inputsplit(strInput, '\n')
slopes = [(1,1), (1,3), (1,5), (1,7), (2,1)]
print(do1(splitInput))
print(do2(splitInput, slopes))
do()<file_sep>/2020/day21.py
from HelperFunctions import inputsplit
import re
from copy import deepcopy
def do1(puzzleInput):
completeListOfIngredients, possibleAllergens = getIngredients(puzzleInput)
badIngredients = extractBadIngredients(possibleAllergens)
goodIngredients = countGoodIngredients(completeListOfIngredients, badIngredients)
return goodIngredients
def do2(puzzleInput):
_, possibleAllergens = getIngredients(puzzleInput)
badIngredients = extractBadIngredients(possibleAllergens)
canonical = getCanonicalDangerousList(badIngredients)
return canonical
def getCanonicalDangerousList(badIngredients):
sortedAllergens = sorted(list(badIngredients.keys()))
result = ''
for allergen in sortedAllergens:
result += badIngredients[allergen]
if allergen != sortedAllergens[-1]:
result += ','
return result
def getIngredients(puzzleInput):
allergensInIngredients = {}
completeListOfIngredients = []
for line in puzzleInput:
match = re.match('(.+) \(contains (.+)\)', line)
if match:
allIngredients = match.groups()[0].split(' ')
completeListOfIngredients += allIngredients
ingredients = set(allIngredients)
allergens = match.groups()[1].replace(' ', '').split(',')
for allergen in allergens:
if allergen not in allergensInIngredients.keys():
allergensInIngredients[allergen] = ingredients
else:
allergensInIngredients[allergen] = allergensInIngredients[allergen] & ingredients
return completeListOfIngredients, allergensInIngredients
def countGoodIngredients(ingredients, badIngredients):
for value in badIngredients.values():
ingredients = [x for x in ingredients if x != value]
return len(ingredients)
def extractBadIngredients(allergens):
solution = {}
while (len(solution.keys()) != len(allergens.keys())):
for allergen,ingredients in allergens.items():
if len(ingredients) == 1:
solution[allergen] = ingredients.pop()
for value in allergens.values():
value.discard(solution[allergen])
return solution
def do():
with open ('Input/day21.txt') as f:
strInput = f.read()
splitInput = inputsplit(strInput, '\n')
print(do1(splitInput))
print(do2(splitInput))
do()<file_sep>/2020/day1.py
from HelperFunctions import inputsplit
from HelperFunctions import convertToInt
def do1(intInput):
multiply = set([x*y for x in intInput for y in intInput if (x + y) == 2020])
return multiply
def do2(intInput):
multiply = set([x*y*z for x in intInput for y in intInput for z in intInput if (x + y + z) == 2020])
return multiply
def do():
with open ('Input/day1.txt') as f:
strInput = f.read()
splitInput = inputsplit(strInput, '\n')
intInput = convertToInt(splitInput)
print(do1(intInput))
print(do2(intInput))
do()<file_sep>/2019/day14.py
from collections import namedtuple
import re
input = """1 ZQVND => 2 MBZM
2 KZCVX, 1 SZBQ => 7 HQFB
1 PFSQF => 9 RSVN
2 PJXQB => 4 FSNZ
20 JVDKQ, 2 LSQFK, 8 SDNCK, 1 MQJNV, 13 LBTV, 3 KPBRX => 5 QBPC
131 ORE => 8 WDQSL
19 BRGJH, 2 KNVN, 3 CRKW => 9 MQJNV
16 DNPM, 1 VTVBF, 11 JSGM => 1 BWVJ
3 KNVN, 1 JQRML => 7 HGQJ
1 MRQJ, 2 HQFB, 1 MQJNV => 5 VQLP
1 PLGH => 5 DMGF
12 DMGF, 3 DNPM, 1 CRKW => 1 CLML
1 JSGM, 1 RSVN => 5 TMNKH
1 RFJLG, 3 CFWC => 2 ZJMC
1 BRGJH => 5 KPBRX
1 SZBQ, 17 GBVJF => 4 ZHGL
2 PLGH => 5 CFWC
4 FCBZS, 2 XQWHB => 8 JSGM
2 PFSQF => 2 KNVN
12 CRKW, 9 GBVJF => 1 KRCB
1 ZHGL => 8 PJMFP
198 ORE => 2 XQWHB
2 BWVJ, 7 CFWC, 17 DPMWN => 3 KZCVX
4 WXBF => 6 JVDKQ
2 SWMTK, 1 JQRML => 7 QXGZ
1 JSGM, 1 LFSFJ => 4 LSQFK
73 KNVN, 65 VQLP, 12 QBPC, 4 XGTL, 10 SWMTK, 51 ZJMC, 4 JMCPR, 1 VNHT => 1 FUEL
1 BWVJ, 7 MBZM => 5 JXZT
10 CFWC => 2 DPMWN
13 LQDLN => 3 LBTV
1 PFZW, 3 LQDLN => 5 PJXQB
2 RSVN, 2 PFSQF => 5 CRKW
1 HGQJ, 3 SMNGJ, 36 JXZT, 10 FHKG, 3 KPBRX, 2 CLML => 3 JMCPR
126 ORE => 4 FCBZS
1 DNPM, 13 MBZM => 5 PLGH
2 XQWHB, 10 FCBZS => 9 LFSFJ
1 DPMWN => 9 PFZW
1 ZJMC, 3 TMNKH => 2 SWMTK
7 TZCK, 1 XQWHB => 5 ZQVND
4 CFWC, 1 ZLWN, 5 RSVN => 2 WXBF
1 BRGJH, 2 CLML => 6 LQDLN
26 BWVJ => 2 GBVJF
16 PJXQB, 20 SDNCK, 3 HQFB, 7 QXGZ, 2 KNVN, 9 KZCVX => 8 XGTL
8 PJMFP, 3 BRGJH, 19 MRQJ => 5 SMNGJ
7 DNPM => 2 SZBQ
2 JQRML, 14 SDNCK => 8 FHKG
1 FSNZ, 6 RFJLG, 2 CRKW => 8 SDNCK
2 CLML, 4 SWMTK, 16 KNVN => 4 JQRML
8 TZCK, 18 WDQSL => 2 PFSQF
1 LSQFK => 8 VTVBF
18 BRGJH, 8 ZHGL, 2 KRCB => 7 VNHT
3 TZCK => 4 DNPM
14 PFZW, 1 PFSQF => 7 BRGJH
21 PLGH, 6 VTVBF, 2 RSVN => 1 ZLWN
149 ORE => 2 TZCK
3 JSGM => 1 RFJLG
4 PFSQF, 4 DMGF => 3 MRQJ"""
Ingredients = namedtuple('Ingredients', 'ingredients result')
usedOres = 0
def do2():
splitInput = input.split('\n')
recipes = getRecipes(splitInput)
stuff = {}
stuff['ORE'] = 1000000000000
repeat = True
while repeat:
repeat, sumOfFuel = makeStuff(recipes, stuff, 'FUEL', 2)
return sumOfFuel
def do():
splitInput = input.split('\n')
recipes = getRecipes(splitInput)
stuff = {}
makeStuff(recipes, stuff, 'FUEL', 1)
def getRecipes(splitInput):
recipes = {}
for line in splitInput:
match = re.findall('([0-9]+) ([A-Z]+)', line)
ingredients = {}
for i in range(len(match) - 1):
ingredients[match[i][1]] = int(match[i][0])
recipes[match[-1][1]] = Ingredients(result=int(match[-1][0]), ingredients=ingredients)
return recipes
def makeStuff(recipes, stuff, name, part):
global usedOres
creationWorks = False
while not creationWorks:
allIngredients = True
for ingredient in recipes[name].ingredients.keys():
numberNeeded = recipes[name].ingredients[ingredient]
if stuff.get(ingredient, 0) < numberNeeded:
allIngredients = False
if ingredient == 'ORE':
if part == 2:
return [False, stuff.get('FUEL', 0)]
else:
neededOres = numberNeeded - stuff.get('ORE', 0)
usedOres += neededOres
stuff['ORE'] = stuff.get('ORE', 0) + neededOres
else:
repeat, sum = makeStuff(recipes, stuff, ingredient, part)
if not repeat:
return [False, stuff.get('FUEL', 0)]
if allIngredients:
creationWorks = True
stuff[name] = stuff.get(name, 0) + recipes[name].result
for ingredient in recipes[name].ingredients.keys():
numberNeeded = recipes[name].ingredients[ingredient]
stuff[ingredient] -= numberNeeded
return [True, stuff.get('FUEL', 0)]
do()
print(usedOres)
print(do2())
<file_sep>/2021/day2.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
def do1(splitInput):
horizontal = 0
depth = 0
for line in splitInput:
direction,value = line.split(' ')
value = int(value)
match direction:
case 'forward':
horizontal += value
case 'up':
depth -= value
case 'down':
depth += value
return horizontal * depth
def do2(splitInput):
horizontal = 0
depth = 0
aim = 0
for line in splitInput:
direction,value = line.split(' ')
value = int(value)
match direction:
case 'forward':
horizontal += value
depth += aim * value
case 'up':
aim -= value
case 'down':
aim += value
return horizontal * depth
def do():
strInput = readInputFile(2)
splitInput = strInput.split('\n')
print(do1(splitInput))
print(do2(splitInput))
print('done')
do()<file_sep>/2021/day22.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
import re
def do1(splitInput):
points = set()
for line in splitInput:
onorOff,xmin,xmax,ymin,ymax,zmin,zmax = getLine(line)
if xmin < -50 or xmax > 50 or ymin < -50 or ymax > 50 or zmin < -50 or zmax > 50:
continue
for x in range(xmin, xmax + 1):
for y in range(ymin, ymax + 1):
for z in range(zmin, zmax + 1):
match onorOff:
case 'on':
points.add((x,y,z))
case 'off':
if (x,y,z) in points:
points.remove((x,y,z))
case _:
print('missing implementation')
return len(points)
def do2(splitInput):
processed = []
for line in splitInput:
onorOff,xmin,xmax,ymin,ymax,zmin,zmax = getLine(line)
cube = (xmin,xmax,ymin,ymax,zmin,zmax)
newCubes = []
for otherCube,activation in processed:
intersectionCube = intersectCubes(cube, otherCube)
if intersectionCube:
if activation == 'on':
newActivation = 'off'
else:
newActivation = 'on'
newCubes.append((intersectionCube, newActivation))
if onorOff == 'on':
processed.append((cube, onorOff))
processed += newCubes
on = 0
for cube,activation in processed:
volume = getCubeVolume(cube)
if activation == 'on':
on += volume
else:
on -= volume
return on
def getCubeVolume(cube):
if not cube:
return 0
xmin,xmax,ymin,ymax,zmin,zmax = cube
return abs(xmax + 1 - xmin) * abs(ymax + 1 - ymin) * abs(zmax + 1 - zmin)
def intersectCubes(cube1, cube2):
xmin1,xmax1,ymin1,ymax1,zmin1,zmax1 = cube1
xmin2,xmax2,ymin2,ymax2,zmin2,zmax2 = cube2
if xmin1 < xmin2:
if xmin2 > xmax1:
return []
else:
if xmin1 > xmax2:
return []
if ymin1 < ymin2:
if ymin2 > ymax1:
return []
else:
if ymin1 > ymax2:
return []
if zmin1 < zmin2:
if zmin2 > zmax1:
return []
else:
if zmin1 > zmax2:
return []
ixmin = max(xmin1, xmin2)
iymin = max(ymin1, ymin2)
izmin = max(zmin1, zmin2)
ixmax = min(xmax1, xmax2)
iymax = min(ymax1, ymax2)
izmax = min(zmax1, zmax2)
return [ixmin,ixmax,iymin,iymax,izmin,izmax]
def getLine(line):
match = re.findall('(on|off) x=(-?[0-9]+)\.\.(-?[0-9]+)',line)
onOrOff,xmin,xmax = match[0]
xmin,xmax = convertToInt((xmin,xmax))
match = re.findall('y=(-?[0-9]+)\.\.(-?[0-9]+)',line)
ymin,ymax = convertToInt(match[0])
match = re.findall('z=(-?[0-9]+)\.\.(-?[0-9]+)',line)
zmin,zmax = convertToInt(match[0])
return onOrOff,xmin,xmax,ymin,ymax,zmin,zmax
def do():
strInput = readInputFile(22)
splitInput = strInput.split('\n')
print(do1(splitInput))
print(do2(splitInput))
print('done')
do()<file_sep>/2020/day19.py
from HelperFunctions import inputsplit
import re
from HelperFunctions import convertToInt
from copy import deepcopy
def do1(puzzleInput):
rules = getRules(puzzleInput[0])
messages = puzzleInput[1].split('\n')
result = checkMessages(messages, rules, 0)
return result
def do2(puzzleInput):
rules = getRules(puzzleInput[0])
messages = puzzleInput[1].split('\n')
result = checkMessages2(messages, rules, 0)
return result
def getRules(puzzleInput):
rules = {}
missing = {}
splitInput = puzzleInput.split('\n')
newInput = deepcopy(splitInput)
for rule in splitInput:
match = re.match('([0-9]+): "(.+)"', rule)
if match:
rules[int(match.groups()[0])] = [match.groups()[1]]
newInput.remove(rule)
else:
match = re.match('([0-9]+): (.+)', rule)
missing[int(match.groups()[0])] = match.groups()[1]
ls = deepcopy(missing)
for key in ls.keys():
getRule(key, rules, missing)
return rules
def getRule(rule, rules, missing):
if rule in rules.keys():
return rules[rule]
match = re.fullmatch('(([ ]?[0-9]+)+)', missing[rule])
if match:
subrules = convertToInt(match.groups()[0].split(' '))
result = getResult(subrules, rules, missing)
rules[rule] = result
else:
match = re.fullmatch('(([ ]?[0-9]+)+) \| (([ ]?[0-9]+)+)', missing[rule])
if match:
alternatives = []
alternatives.append(match.groups()[0])
alternatives.append(match.groups()[2])
completeResult = []
for alternative in alternatives:
subrules = convertToInt(alternative.split(' '))
result = getResult(subrules, rules, missing)
completeResult += result
rules[rule] = completeResult
del missing[rule]
return rules[rule]
def getResult(subrules, rules, missing):
result = []
for subrule in subrules:
alternatives = getRule(subrule, rules, missing)
if not result:
result = deepcopy(alternatives)
else:
tmp = []
for partResult in result:
for alternative in alternatives:
tmp.append(partResult + alternative)
result = tmp
return result
def checkMessages(messages, rules, ruleNumber):
count = 0
for message in messages:
if message in rules[ruleNumber]:
count += 1
return count
def checkMessages2(messages, rules, ruleNumber):
blobSize = len(rules[42][0])
count = 0
for message in messages:
if message in rules[ruleNumber]:
count += 1
else:
count42 = 0
count31 = 0
successful = True
for position in range(0, len(message) - blobSize + 1, blobSize):
messageSlice = message[position:(position + blobSize)]
if messageSlice in rules[42]:
count42 += 1
if count31:
successful = False
break
elif messageSlice in rules[31]:
count31 += 1
if successful and count31 and count42 - count31 > 0:
count += 1
return count
def do():
with open ('Input/day19.txt') as f:
strInput = f.read()
splitInput = inputsplit(strInput, '\n\n')
print(do1(splitInput))
print(do2(splitInput))
do()<file_sep>/2021/day14.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
import re
from collections import Counter
from copy import deepcopy
from itertools import pairwise
def do1(start, rules):
ruleSet = getRules(rules)
occurences = insert(start, ruleSet, 10)
mostCommon = max(occurences.values())
leastCommon = min(occurences.values())
return mostCommon - leastCommon
def do2(start, rules):
ruleSet = getRules(rules)
occurences = insert(start, ruleSet, 40)
mostCommon = max(occurences.values())
leastCommon = min(occurences.values())
return mostCommon - leastCommon
def getRules(rules):
ruleSet = {}
for rule in rules:
match = re.fullmatch('([A-Z]+) -> ([A-Z])', rule)
if match:
ruleSet[(match.groups()[0][0], match.groups()[0][1])] = match.groups()[1]
return ruleSet
def insert(start, ruleSet, steps):
occurences = Counter()
pairOccurences = Counter()
for x in start:
occurences[x] += 1
pairs = pairwise(start)
for pair in pairs:
pairOccurences[pair] += 1
newPairOccurences = deepcopy(pairOccurences)
for _ in range(steps):
pairOccurences = deepcopy(newPairOccurences)
for (x,y),quantity in pairOccurences.items():
if quantity == 0:
continue
newElement = ruleSet[(x,y)]
occurences[newElement] += quantity
newPairOccurences[(x,newElement)] += quantity
newPairOccurences[(newElement, y)] += quantity
newPairOccurences[(x,y)] -= quantity
return occurences
def do():
strInput = readInputFile(14)
start,rules = strInput.split('\n\n')
rules = rules.split('\n')
print(do1(start, rules))
print(do2(start, rules))
print('done')
do()<file_sep>/2021/day24.py
from functools import cache
inputDict = {1: 2, 2: 3, 3: 4, 4: 5, 5: 6, 6: 7, 7: 8, 8: 9, 9: 10}
def do1():
global inputDict
k = [1, 10, 2, 5, 6, 0, 16, 12, 15, 7, 6, 5, 6, 15]
special = {3: 10, 7: 11, 8: 7, 10: 13, 11: 0, 12: 11, 13: 0, }
for position in range(1,14):
newDict = inputDict.copy()
for oldW, z in inputDict.items():
if position in special.keys():
w = (z % 26) - special[position]
if w < 1 or w > 9:
del newDict[oldW]
continue
znew = z // 26
newDict[oldW * 10 + w] = znew
else:
for w in range(1,10):
znew = 26 * z + w + k[position]
newDict[oldW * 10 + w] = znew
del newDict[oldW]
inputDict = newDict.copy()
possibleValues = [x for x in inputDict.keys() if inputDict[x] == 0]
return max(possibleValues)
def do2():
global inputDict
possibleValues = [x for x in inputDict.keys() if inputDict[x] == 0]
return min(possibleValues)
def do():
print(do1())
print(do2())
print('done')
do()<file_sep>/2020/day13.py
from HelperFunctions import inputsplit
def do1(earliest, puzzleInput):
bestBusID = 0
leastWaitingTime = 100000000000
for bus in puzzleInput:
if bus == 'x':
continue
busDepartTime = 0
busID = int(bus)
while busDepartTime < earliest:
busDepartTime += busID
if (busDepartTime - earliest) < leastWaitingTime:
leastWaitingTime = busDepartTime - earliest
bestBusID = busID
return bestBusID * leastWaitingTime
def do2(puzzleInput):
maxBus = max([x for x in puzzleInput if x != 'x'])
maxBusIndex = puzzleInput.index(maxBus)
maxBus = int(maxBus)
departTime = 487905974205117 - maxBus# 100000000000481-maxBusIndex
works = False
while not works:
works = True
departTime += maxBus
for i in range(len(puzzleInput)):
bus = puzzleInput[i]
if bus == 'x':
continue
busID = int(bus)
if (departTime + i) % busID != 0:
works = False
break
return departTime
def do():
with open ('Input/day13.txt') as f:
strInput = f.read()
splitInput = inputsplit(strInput, ',')
earliest = 1007125
print(do1(earliest, splitInput))
print(do2(splitInput))
do()
<file_sep>/2022/day23.py
from collections import namedtuple
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
directions = ['n', 's', 'w', 'e']
Neighbors = namedtuple('Neighbors', ['NW', 'N', 'NE', 'W', 'E', 'SW', 'S', 'SE'])
def do1(splitInput):
elves = extractElves(splitInput)
directionPos = 0
for _ in range(10):
newElves = {}
for elf in elves:
neighbors = getNeighbors(elf, elves)
if not neighbors.N and not neighbors.NE and not neighbors.NW and not neighbors.W and not neighbors.E and not neighbors.SW and not neighbors.S and not neighbors.SE:
continue
for i in range(4):
direction = directions[(directionPos + i) % 4]
match direction:
case 'n':
if not neighbors.N and not neighbors.NE and not neighbors.NW:
newElves[elf] = (elf[0] - 1, elf[1])
break
case 's':
if not neighbors.S and not neighbors.SE and not neighbors.SW:
newElves[elf] = (elf[0] + 1, elf[1])
break
case 'w':
if not neighbors.W and not neighbors.NW and not neighbors.SW:
newElves[elf] = (elf[0], elf[1] - 1)
break
case 'e':
if not neighbors.E and not neighbors.NE and not neighbors.SE:
newElves[elf] = (elf[0], elf[1] + 1)
break
directionPos = (directionPos + 1) % 4
newPositions = list(newElves.values())
for elf,newPos in newElves.items():
if newPositions.count(newPos) == 1:
elves.remove(elf)
elves.append(newPos)
xMin = min([elf[0] for elf in elves])
xMax = max([elf[0] for elf in elves])
yMin = min([elf[1] for elf in elves])
yMax = max([elf[1] for elf in elves])
freeTiles = 0
for x in range(xMin, xMax + 1):
for y in range(yMin, yMax + 1):
if (x,y) not in elves:
freeTiles += 1
return freeTiles
def do2(splitInput):
elves = extractElves(splitInput)
directionPos = 0
round = 0
while True:
round += 1
newElves = {}
for elf in elves:
neighbors = getNeighbors(elf, elves)
if not neighbors.N and not neighbors.NE and not neighbors.NW and not neighbors.W and not neighbors.E and not neighbors.SW and not neighbors.S and not neighbors.SE:
continue
for i in range(4):
direction = directions[(directionPos + i) % 4]
match direction:
case 'n':
if not neighbors.N and not neighbors.NE and not neighbors.NW:
newElves[elf] = (elf[0] - 1, elf[1])
break
case 's':
if not neighbors.S and not neighbors.SE and not neighbors.SW:
newElves[elf] = (elf[0] + 1, elf[1])
break
case 'w':
if not neighbors.W and not neighbors.NW and not neighbors.SW:
newElves[elf] = (elf[0], elf[1] - 1)
break
case 'e':
if not neighbors.E and not neighbors.NE and not neighbors.SE:
newElves[elf] = (elf[0], elf[1] + 1)
break
directionPos = (directionPos + 1) % 4
newPositions = list(newElves.values())
if not newPositions:
return round
for elf,newPos in newElves.items():
if newPositions.count(newPos) == 1:
elves.remove(elf)
elves.append(newPos)
def extractElves(splitInput):
elves = []
for lineNumber,line in enumerate(splitInput):
for position,tile in enumerate(line):
if tile == '#':
elves.append((lineNumber,position))
return elves
def getNeighbors(elf, elves):
elfX,elfY = elf
neighbors = []
for xDiff in [-1, 0, 1]:
for yDiff in [-1, 0, 1]:
if xDiff == 0 and yDiff == 0:
continue
if (elfX + xDiff, elfY + yDiff) in elves:
neighbors.append(True)
else:
neighbors.append(False)
return Neighbors(NW=neighbors[0],N=neighbors[1],NE=neighbors[2],W=neighbors[3],E=neighbors[4],SW=neighbors[5],S=neighbors[6],SE=neighbors[7])
def do():
strInput = readInputFile(23)
#strInput = readExampleInput(23)
splitInput = strInput.split('\n')
print(do1(splitInput))
print(do2(splitInput))
print('done')
do()<file_sep>/2021/day25.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
from copy import deepcopy
def do1(splitInput):
map = []
for line in splitInput:
newLine = [x for x in line]
map.append(newLine)
mapLength = len(map)
newMap = deepcopy(map)
moving = True
steps = 0
southfacing = True
while moving or not southfacing:
southfacing = not southfacing
moving = False
if not southfacing:
steps += 1
for lineNumber,line in enumerate(map):
lineLength = len(line)
for position,value in enumerate(line):
match value:
case '.':
continue
case 'v':
if southfacing:
neighborLine = (lineNumber + 1) % mapLength
if map[neighborLine][position] == '.':
newMap[neighborLine][position] = 'v'
newMap[lineNumber][position] = '.'
moving = True
case '>':
if not southfacing:
neighborPos = (position + 1) % lineLength
if line[neighborPos] == '.':
newMap[lineNumber][neighborPos] = '>'
newMap[lineNumber][position] = '.'
moving = True
map = deepcopy(newMap)
return steps
def do2():
return 'Nothing to do'
def do():
strInput = readInputFile(25)
splitInput = strInput.split('\n')
print(do1(splitInput))
print(do2())
print('done')
do()<file_sep>/2020/day8.py
from HelperFunctions import inputsplit
def do1(puzzleInput):
success, acc = execute(puzzleInput)
if not success:
return acc
else:
return 'error'
def do2(oldPuzzleInput):
replacePosition = -1
while (replacePosition < len(oldPuzzleInput)):
replacePosition += 1
puzzleInput = oldPuzzleInput.copy()
if not replaceInstruction(replacePosition, puzzleInput):
continue
success, acc = execute(puzzleInput)
if success:
return acc
return 'error'
def execute(puzzleInput):
acc = 0
visited = [0 for x in range(len(puzzleInput))]
position = 0
while True:
if position == len(puzzleInput):
return [True, acc]
if visited[position] == 1:
return [False, acc]
instructions = puzzleInput[position].split(' ')
if instructions[0] == 'nop':
visited[position] = 1
position += 1
continue
if instructions[0] == 'acc':
visited[position] = 1
acc += int(instructions[1])
position += 1
continue
if instructions[0] == 'jmp':
visited[position] = 1
position += int(instructions[1])
continue
def replaceInstruction(position, puzzleInput):
if 'acc' in puzzleInput[position]:
return False
else:
instructions = puzzleInput[position].split(' ')
if instructions[0] == 'jmp':
puzzleInput[position] = 'nop ' + instructions[1]
else:
puzzleInput[position] = 'jmp ' + instructions[1]
return True
def do():
with open ('Input/day8.txt') as f:
strInput = f.read()
splitInput = inputsplit(strInput, '\n')
print(do1(splitInput))
print(do2(splitInput))
do()<file_sep>/2022/day12.py
from collections import defaultdict
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
def do1(strInput):
distances = defaultdict(lambda: 1000)
unvisited = []
visited = []
startingPos,endPos,heightMap = createHeightMap(strInput.split('\n'))
distances[startingPos] = 0
unvisited.append((startingPos))
findShortestPath(heightMap, visited, unvisited, distances)
return distances[endPos]
def do2(strInput):
_,endPos,heightMap = createHeightMap(strInput.split('\n'))
startingPositions = []
for lineNumber,line in enumerate(heightMap):
for index,position in enumerate(line):
if position == ord('a'):
startingPositions.append((lineNumber,index))
distancesToDestination = []
for startingPosition in startingPositions:
unvisited = []
visited = []
distances = defaultdict(lambda: 1000)
distances[startingPosition] = 0
unvisited.append(startingPosition)
findShortestPath(heightMap, visited, unvisited, distances)
distancesToDestination.append(distances[endPos])
return min(distancesToDestination)
def createHeightMap(map):
for lineNumber,line in enumerate(map):
for index,position in enumerate(line):
if position == 'S':
startingPos = (lineNumber,index)
elif position == 'E':
endPos = (lineNumber, index)
heightMap = [[ord(x) for x in y] for y in map]
heightMap[startingPos[0]][startingPos[1]] = ord('a')
heightMap[endPos[0]][endPos[1]] = ord('z')
return (startingPos, endPos, heightMap)
def findShortestPath(heightMap, visited, unvisited, distances):
while unvisited:
currentPos = None
currentDistance = 0
for position in unvisited:
if currentPos == None:
currentPos = position
currentDistance = distances[position]
elif distances[position] < distances[currentPos]:
currentPos = position
currentDistance = distances[position]
neighbors = getPossibleNeighbors(currentPos, heightMap)
for neighbor in neighbors:
if neighbor not in visited and neighbor not in unvisited:
unvisited.append(neighbor)
if distances[neighbor] > currentDistance + 1:
distances[neighbor] = currentDistance + 1
visited.append(currentPos)
unvisited.remove(currentPos)
def getPossibleNeighbors(position, map):
possibleNeighbors = []
line,index = position
currentHeight = map[line][index]
if line - 1 >= 0 and map[line-1][index] <= currentHeight + 1:
possibleNeighbors.append((line-1,index))
if line + 1 < len(map) and map[line+1][index] <= currentHeight + 1:
possibleNeighbors.append((line+1,index))
if index + 1 < len(map[0]) and map[line][index+1] <= currentHeight + 1:
possibleNeighbors.append((line,index+1))
if index - 1 >= 0 and map[line][index-1] <= currentHeight + 1:
possibleNeighbors.append((line,index-1))
return possibleNeighbors
def do():
strInput = readInputFile(12)
print(do1(strInput))
print(do2(strInput))
print('done')
do()<file_sep>/2022/day8.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
def do1(splitInput):
visibleTrees = 0
for linePos,line in enumerate(splitInput):
for position,_ in enumerate(line):
if onlySmallerTrees(linePos,position,splitInput):
visibleTrees += 1
return visibleTrees
def do2(splitInput):
scenicScores = []
for linePos,line in enumerate(splitInput):
for position,_ in enumerate(line):
scenicScores.append(calculateScenicScore(linePos,position,splitInput))
return max(scenicScores)
def onlySmallerTrees(linePos, position, map):
tree = map[linePos][position]
#left
otherTrees = [x for x in map[linePos][0:position] if x >= tree]
if not otherTrees:
return True
#right
otherTrees = [x for x in map[linePos][position + 1:] if x >= tree]
if not otherTrees:
return True
#up
otherTrees = [x[position] for x in map[0:linePos] if x[position] >= tree]
if not otherTrees:
return True
#down
otherTrees = [x[position] for x in map[linePos+1:] if x[position] >= tree]
if not otherTrees:
return True
def calculateScenicScore(linePos,position,map):
tree = map[linePos][position]
#right
scoreRight = 0
for i in range(position + 1, len(map[linePos])):
scoreRight += 1
if map[linePos][i] >= tree:
break
#left
scoreLeft = 0
for i in range(position - 1, -1, -1):
scoreLeft += 1
if map[linePos][i] >= tree:
break
#down
scoreDown = 0
for i in range(linePos + 1, len(map)):
scoreDown += 1
if map[i][position] >= tree:
break
#up
scoreUp = 0
for i in range(linePos - 1, -1, -1):
scoreUp += 1
if map[i][position] >= tree:
break
return scoreRight * scoreLeft * scoreDown * scoreUp
def do():
strInput = readInputFile(8)
splitInput = strInput.split('\n')
print(do1(splitInput))
print(do2(splitInput))
print('done')
do()<file_sep>/2022/day13.py
import ast
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
def do1(splitInput):
rightOrderPairs = 0
for index,pair in enumerate(splitInput):
left,right = pair.split('\n')
left = ast.literal_eval(left)
right = ast.literal_eval(right)
successful,result = compare(left,right)
if successful and result:
rightOrderPairs += index + 1
return rightOrderPairs
def do2(strInput):
result = []
strInput = strInput.replace('\n\n', '\n')
splitInput = strInput.split('\n')
splitInput.append('[[2]]')
splitInput.append('[[6]]')
first = ast.literal_eval(splitInput[0])
result.append(first)
for line in splitInput[1:]:
inserted = False
new = ast.literal_eval(line)
for index,old in enumerate(result):
successful,rightOrder = compare(old,new)
if successful and not rightOrder:
result.insert(index,new)
inserted = True
break
if not inserted:
result.append(new)
return (result.index([[2]]) + 1) * (result.index([[6]]) + 1)
def compare(left,right):
leftLength = len(left)
rightLength = len(right)
i = -1
while True:
i += 1
if leftLength < i + 1 and rightLength >= i + 1:
return True,True
elif rightLength < i + 1 and leftLength >= i + 1:
return True,False
elif leftLength < i + 1 and rightLength < i + 1:
return False,False
leftPart = left[i]
rightPart = right[i]
if type(leftPart) == type(rightPart):
if type(leftPart) is int:
if leftPart < rightPart:
return True,True
elif rightPart < leftPart:
return True,False
else:
continue
elif type(leftPart) is list:
successful,result = compare(leftPart,rightPart)
if successful:
return True,result
else:
continue
if type(leftPart) is int:
leftPart = [leftPart]
else:
rightPart = [rightPart]
successful,result = compare(leftPart,rightPart)
if successful:
return True,result
else:
continue
def do():
strInput = readInputFile(13)
splitInput = strInput.split('\n\n')
print(do1(splitInput))
print(do2(strInput))
print('done')
do()<file_sep>/2022/day10.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
image = []
def do1(splitInput):
signalStrengths = []
cycle = 0
x = 1
for line in splitInput:
cycle += 1
if cycle == 20 or (cycle-20) % 40 == 0:
signalStrengths.append(x * cycle)
match line.split(' '):
case ['addx', value]:
cycle += 1
if cycle == 20 or (cycle-20) % 40 == 0:
signalStrengths.append(x * cycle)
x = x + int(value)
return sum(signalStrengths)
def do2(splitInput):
spritePos = 1
cycle = 0
for line in splitInput:
cycle += 1
draw(spritePos, cycle)
match line.split(' '):
case ['addx', value]:
cycle += 1
draw(spritePos, cycle)
spritePos += int(value)
for line in image:
print(line)
return 'done'
def draw(spritePos, cycle):
line = int((cycle - 1) / 40)
if len(image) < line + 1:
image.append(['.' for x in range(40)])
position = (cycle - 1) % 40
if position in [spritePos-1,spritePos,spritePos+1]:
image[line][position] = '#'
def do():
strInput = readInputFile(10)
splitInput = strInput.split('\n')
print(do1(splitInput))
print(do2(splitInput))
print('done')
do()<file_sep>/2018/day6.py
input = """118, 274
102, 101
216, 203
208, 251
309, 68
330, 93
91, 179
298, 278
201, 99
280, 272
141, 312
324, 290
41, 65
305, 311
198, 68
231, 237
164, 224
103, 189
216, 207
164, 290
151, 91
166, 250
129, 149
47, 231
249, 100
262, 175
299, 237
62, 288
228, 219
224, 76
310, 173
80, 46
312, 65
183, 158
272, 249
57, 141
331, 191
163, 359
271, 210
142, 137
349, 123
55, 268
160, 82
180, 70
231, 243
133, 353
246, 315
164, 206
229, 97
268, 94"""
import string
import re
def nearest(coordinates, dic):
xpoint,ypoint = coordinates
nearestDistance = 10000
result = 0
for entry in dic:
x,y = dic[entry]
distance = abs(x - xpoint) + abs(y - ypoint)
if distance < nearestDistance:
nearestDistance = distance
result = entry
elif distance == nearestDistance:
result = 0
return result
def nearEnough(coordinates, dic):
xpoint,ypoint = coordinates
maxDistance = 10000
distanceSum = 0
for entry in dic:
x,y = dic[entry]
distanceSum += abs(x - xpoint) + abs(y - ypoint)
if distanceSum >= maxDistance:
return False
return True
def doOne():
splitInput = input.split('\n')
dic ={}
count = 1
for coordinates in splitInput:
dic[count] = [int(x) for x in re.findall('[0-9]+', coordinates)]
count += 1
width, height = 350, 370
matrix = [[0 for x in range(width)] for y in range(height)]
resultdic = {}
for x in range(width):
for y in range(height):
near = nearest([x,y], dic)
matrix[y][x] = near
if near not in resultdic:
resultdic[near] = 0
if x == width - 1 or y == height - 1 or x == 0 or y == 0:
resultdic[near] = -1
if resultdic[near] != -1:
resultdic[near] += 1
return resultdic
def doTwo():
splitInput = input.split('\n')
dic ={}
count = 1
for coordinates in splitInput:
dic[count] = [int(x) for x in re.findall('[0-9]+', coordinates)]
count += 1
width, height = 500, 500
matrix = [[0 for x in range(width)] for y in range(height)]
result = 0
for x in range(width):
for y in range(height):
nearenough = nearEnough([x,y], dic)
if nearenough:
result += 1
return result
print(max(doOne().values()))
print(doTwo())<file_sep>/2020/day23.py
from collections import deque
def do1(puzzleInput):
queue = deque([int(x) for x in puzzleInput])
play(queue, 100)
return cupOrder(queue)
def do2(puzzleInput):
queue = [int(x) for x in puzzleInput] + list(range(10, 1000000+1))
linkedList = play2(queue, 10000000)
return findStars(linkedList)
def cupOrder(queue):
result = ''
first = queue.index(1)
queue.rotate(-(first + 1))
for _ in range(len(queue) - 1):
result += str(queue.popleft())
return result
def findStars(linkedList):
first = linkedList[1]
second = linkedList[first]
return first * second
def play(queue, turns):
maxValue = max(queue)
minValue = min(queue)
for _ in range(1, turns + 1):
currentElement = queue.popleft()
removed = [queue.popleft(), queue.popleft(), queue.popleft()]
newElement = currentElement - 1
if newElement < minValue:
newElement = maxValue
while newElement in removed:
newElement -= 1
if newElement < minValue:
newElement = maxValue
newIndex = queue.index(newElement)
queue.rotate(- (newIndex + 1))
queue.extendleft(removed[::-1])
queue.rotate(newIndex + 1)
queue.append(currentElement)
def play2(queue, turns):
maxValue = max(queue)
minValue = min(queue)
linkedList = {}
for index,item in enumerate(queue[0:-1]):
linkedList[item] = queue[index + 1]
linkedList[queue[-1]] = queue[0]
currentIndex = queue[-1]
for _ in range(1, turns + 1):
currentElement = linkedList[currentIndex]
removed = [linkedList[currentElement], linkedList[linkedList[currentElement]], linkedList[linkedList[linkedList[currentElement]]]]
nextafternextafternext = removed[-1]
newElement = currentElement - 1
if newElement < minValue:
newElement = maxValue
while newElement in removed:
newElement -= 1
if newElement < minValue:
newElement = maxValue
tmp1 = linkedList[newElement]
tmp2 = linkedList[nextafternextafternext]
linkedList[newElement] = linkedList[currentElement]
linkedList[nextafternextafternext] = tmp1
linkedList[currentElement] = tmp2
currentIndex = currentElement
return linkedList
def do():
with open ('Input/day23.txt') as f:
strInput = f.read()
print(do1(strInput))
print(do2(strInput))
do()<file_sep>/2022/day11.py
from copy import deepcopy
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
import re
class Monkey:
def __init__(self, items, operation, value, testMethod, throwIfTrue, throwIfFalse):
self.items = items
self.operation = operation
self.value = value
self.testMethod = testMethod
self.throwIfTrue = throwIfTrue
self.throwIfFalse = throwIfFalse
self.inspectedItems = 0
def addItem(self, item):
self.items.append(item)
originalMonkeys = []
def multiply(value, old):
return old * value
def add(value, old):
return old + value
def multiplyWithItself(old,value):
return old * old
def do1(splitInput):
createMonkeys(splitInput)
monkeys = deepcopy(originalMonkeys)
for _ in range(20):
monkeyIndex = 0
for _ in range(len(monkeys)):
currentMonkey = monkeys[monkeyIndex]
while currentMonkey.items:
currentMonkey.inspectedItems += 1
item = currentMonkey.items.pop(0)
item = currentMonkey.operation(value=currentMonkey.value, old=item)
item = int(item / 3)
if item % currentMonkey.testMethod == 0:
monkeys[currentMonkey.throwIfTrue].addItem(item)
else:
monkeys[currentMonkey.throwIfFalse].addItem(item)
monkeyIndex += 1
inspectedItems = [x.inspectedItems for x in monkeys]
inspectedItems.sort(reverse=True)
return inspectedItems[0] * inspectedItems[1]
def do2(splitInput):
monkeys = deepcopy(originalMonkeys)
divisor = 1
for monkey in monkeys:
divisor *= monkey.testMethod
for _ in range(10000):
monkeyIndex = 0
for _ in range(len(monkeys)):
currentMonkey = monkeys[monkeyIndex]
while currentMonkey.items:
currentMonkey.inspectedItems += 1
item = currentMonkey.items.pop(0)
item = currentMonkey.operation(value=currentMonkey.value, old=item)
item = item - (int(item / divisor) * divisor)
if item % currentMonkey.testMethod == 0:
monkeys[currentMonkey.throwIfTrue].addItem(item)
else:
monkeys[currentMonkey.throwIfFalse].addItem(item)
monkeyIndex += 1
inspectedItems = [x.inspectedItems for x in monkeys]
inspectedItems.sort(reverse=True)
return inspectedItems[0] * inspectedItems[1]
def createMonkeys(splitInput):
for monkey in splitInput:
lines = monkey.split('\n')
startingItems = [int(x[0:2]) for x in lines[1].split(' ')[4:]]
match lines[2].split(' '):
case [*stuff, '*', 'old']:
operation = multiplyWithItself
value = 0
case [*stuff, '*', value]:
operation = multiply
value = int(value)
case [*stuff, '+', value]:
operation = add
value = int(value)
matchTest = re.match(' Test: divisible by (\d+)', lines[3])
testMethod = int(matchTest.groups()[0])
throwIfTrue = int(lines[4][-1])
throwIfFalse = int(lines[5][-1])
originalMonkeys.append(Monkey(startingItems, operation, value, testMethod, throwIfTrue, throwIfFalse))
def do():
strInput = readInputFile(11)
splitInput = strInput.split('\n\n')
print(do1(splitInput))
print(do2(splitInput))
print('done')
do()<file_sep>/2022/day14.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
def do1(splitInput):
map = buildWalls(splitInput)
sand = set()
rested,restingPos = letTheSandFall(map, sand)
while rested:
sand.add(restingPos)
rested,restingPos = letTheSandFall(map, sand)
return len(sand)
def do2(splitInput):
map = buildWalls(splitInput)
sand = set()
highestY = max([x[1] for x in map])
# add floor
for x in range(-1000, 1000+1):
map.add((x, highestY + 2))
rested,restingPos = letTheSandFall(map, sand)
while rested:
sand.add(restingPos)
if restingPos == (500,0):
break
rested,restingPos = letTheSandFall(map, sand)
return len(sand)
def buildWalls(splitInput):
map = set()
for line in splitInput:
parts = line.split(' -> ')
for i in range(1, len(parts)):
startX,startY = [int(x) for x in parts[i].split(',')]
endX,endY = [int(x) for x in parts[i-1].split(',')]
if startY == endY:
x = sorted([startX, endX])
for j in range(x[0], x[1] + 1):
map.add((j, startY))
else:
y = sorted([startY, endY])
for j in range(y[0], y[1] + 1):
map.add((startX, j))
return map
def letTheSandFall(map, sand):
boundaryLeft = min([x[0] for x in map])
boundaryRight = max([x[0] for x in map])
sandStart = (500,0)
position = sandStart
while True:
newPosition = (position[0], position[1] + 1)
if newPosition in map or newPosition in sand:
# diagonally left
if (newPosition[0] - 1, newPosition[1]) in map or (newPosition[0] - 1, newPosition[1]) in sand:
# diagonally right
if (newPosition[0] + 1, newPosition[1]) in map or (newPosition[0] + 1, newPosition[1]) in sand:
return True,position
else:
position = (position[0] + 1, position[1] + 1)
if position[0] > boundaryRight:
return False,(0,0)
continue
else:
position = (position[0] - 1, position[1] + 1)
if position[0] < boundaryLeft:
return False,(0,0)
continue
else:
position = newPosition
def do():
strInput = readInputFile(14)
splitInput = strInput.split('\n')
print(do1(splitInput))
print(do2(splitInput))
print('done')
do()<file_sep>/2022/day18.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
def do1(splitInput):
cubes = extractCubes(splitInput)
openSides = 0
for x,y,z in cubes:
sides = 6
for oX,oY,oZ in cubes:
if oX == x and oY == y and abs(oZ - z) == 1:
sides -= 1
elif oX == x and oZ == z and abs(oY - y) == 1:
sides -= 1
elif oY == y and oZ == z and abs(oX - x) == 1:
sides -= 1
openSides += sides
return openSides
def do2(splitInput):
cubes = extractCubes(splitInput)
maxX = max(x for x,y,z in cubes) + 1
maxY = max(y for x,y,z in cubes) + 1
maxZ = max(z for x,y,z in cubes) + 1
sides = 0
newAir = set()
newAir.add((maxX,maxY,maxZ))
visitedAir = set()
while len(newAir) > 0:
cube = newAir.pop()
cubeNeighbors,airNeighbors = getNeighbors(cube,cubes,maxX,maxY,maxZ,visitedAir)
sides += len(cubeNeighbors)
newAir = newAir.union(airNeighbors)
visitedAir.add(cube)
return sides
def extractCubes(splitInput):
cubes = set()
for line in splitInput:
cube = [int(x) for x in line.split(',')]
cubes.add((cube[0], cube[1], cube[2]))
return cubes
def getNeighbors(cube, cubes, maxX, maxY, maxZ, visitedAir):
cubeNeighbors = set()
airNeighbors = set()
cubeX,cubeY,cubeZ = cube
if cubeX - 1 >= -1:
if (cubeX - 1, cubeY, cubeZ) in cubes:
cubeNeighbors.add((cubeX - 1, cubeY, cubeZ))
elif (cubeX - 1, cubeY, cubeZ) not in visitedAir:
airNeighbors.add((cubeX - 1, cubeY, cubeZ))
if cubeX + 1 <= maxX:
if (cubeX + 1, cubeY, cubeZ) in cubes:
cubeNeighbors.add((cubeX + 1, cubeY, cubeZ))
elif (cubeX + 1, cubeY, cubeZ) not in visitedAir:
airNeighbors.add((cubeX + 1, cubeY, cubeZ))
if cubeY - 1 >= -1:
if (cubeX, cubeY - 1, cubeZ) in cubes:
cubeNeighbors.add((cubeX, cubeY - 1, cubeZ))
elif (cubeX, cubeY - 1, cubeZ) not in visitedAir:
airNeighbors.add((cubeX, cubeY - 1, cubeZ))
if cubeY + 1 <= maxY:
if (cubeX, cubeY + 1, cubeZ) in cubes:
cubeNeighbors.add((cubeX, cubeY + 1, cubeZ))
elif (cubeX, cubeY + 1, cubeZ) not in visitedAir:
airNeighbors.add((cubeX, cubeY + 1, cubeZ))
if cubeZ - 1 >= -1:
if (cubeX, cubeY, cubeZ - 1) in cubes:
cubeNeighbors.add((cubeX, cubeY, cubeZ - 1))
elif (cubeX, cubeY, cubeZ - 1) not in visitedAir:
airNeighbors.add((cubeX, cubeY, cubeZ - 1))
if cubeZ + 1 <= maxZ:
if (cubeX, cubeY, cubeZ + 1) in cubes:
cubeNeighbors.add((cubeX, cubeY, cubeZ + 1))
elif (cubeX, cubeY, cubeZ + 1) not in visitedAir:
airNeighbors.add((cubeX, cubeY, cubeZ + 1))
return cubeNeighbors,airNeighbors
def do():
strInput = readInputFile(18)
splitInput = strInput.split('\n')
print(do1(splitInput))
print(do2(splitInput))
print('done')
do()<file_sep>/2020/day24.py
from HelperFunctions import inputsplit
from copy import deepcopy
directions = {'e': (1,-1,0), 'w': (-1,1,0), 'se': (0,-1,1), 'sw': (-1,0,1), 'ne': (1,0,-1), 'nw': (0,1,-1)}
def do1(puzzleInput):
tiles = set()
rotate(puzzleInput, tiles)
return len(tiles)
def do2(puzzleInput):
tiles = set()
rotate(puzzleInput, tiles)
newTiles = gameOfLife(tiles, 100)
return len(newTiles)
def rotate(puzzleInput, tiles):
for line in puzzleInput:
tile = (0,0,0)
direction = ''
for thing in line:
direction += thing
if direction in directions:
xStep,yStep,zStep = directions[direction]
x,y,z = tile
tile = (x + xStep, y + yStep, z + zStep)
direction = ''
if tile in tiles:
tiles.remove(tile)
else:
tiles.add(tile)
def gameOfLife(tiles, rounds):
newTiles = deepcopy(tiles)
for _ in range(rounds):
tiles = deepcopy(newTiles)
neighbors = set()
for tile in tiles:
allNeighbors = getNeighbors(tile, tiles)
neighbors = neighbors.union(allNeighbors)
blackNeighbors = countBlackNeighbors(allNeighbors, tiles)
if blackNeighbors == 0 or blackNeighbors > 2:
newTiles.remove(tile)
for neighbor in neighbors:
if neighbor in tiles:
continue
blackNeighbors = countBlackNeighbors(getNeighbors(neighbor, tiles), tiles)
if blackNeighbors == 2:
newTiles.add(neighbor)
return newTiles
def getNeighbors(tile, tiles):
x,y,z = tile
neighbors = set([(x + xStep, y + yStep, z + zStep) for xStep,yStep,zStep in directions.values()])
return neighbors
def countBlackNeighbors(neighbors, tiles):
count = len([neighbor for neighbor in neighbors if neighbor in tiles])
return count
def do():
with open ('Input/day24.txt') as f:
strInput = f.read()
puzzleInput = strInput.split('\n')
print(do1(puzzleInput))
print(do2(puzzleInput))
do()<file_sep>/2021/day17.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
def do1(xRange, yRange):
xMin,xMax = convertToInt(xRange.split(','))
yMin,yMax = convertToInt(yRange.split(','))
maxY = -100000
for xVel in range(1, xMax + 1):
for yVel in range(0, 200):
currentMaxY = fly((0,0), (xVel,yVel), (xMin,xMax), (yMin, yMax))
maxY = max(maxY, currentMaxY)
return maxY
def do2(xRange, yRange):
xMin,xMax = convertToInt(xRange.split(','))
yMin,yMax = convertToInt(yRange.split(','))
landingVels = []
for xVel in range(1, xMax + 1):
for yVel in range(-100, 200):
currentMaxY = fly((0,0), (xVel,yVel), (xMin,xMax), (yMin, yMax))
if currentMaxY != -1000000:
landingVels.append((xVel,yVel))
return len(landingVels)
def step(xPos, yPos, xVel, yVel):
xPos = xPos + xVel
yPos = yPos + yVel
if xVel > 0:
xVel -= 1
elif xVel < 0:
xVel += 1
yVel -= 1
return (xPos, yPos, xVel, yVel)
def fly(position, velocity, targetX, targetY):
maxY = -1000000
x,y = position
xVel,yVel = velocity
yMin,_ = targetY
while True:
x,y,xVel,yVel = step(x, y, xVel, yVel)
maxY = max(maxY, y)
if isInTargetArea((x, y), targetX, targetY):
return maxY
if y < yMin and yVel <= 0:
return -1000000
def isInTargetArea(position, targetX, targetY):
x,y = position
xMin,xMax = targetX
yMin, yMax = targetY
if xMin <= x <= xMax and yMin <= y <= yMax:
return True
return False
def do():
strInput = readInputFile(17)
xRange,yRange = strInput.split('\n')
print(do1(xRange,yRange))
print(do2(xRange,yRange))
print('done')
do()<file_sep>/2021/day20.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
from copy import deepcopy
def do1(enhancement,image):
extendedImage = prepareImage(image, 10)
newImage = imageEnhancement(extendedImage, enhancement, 2)
return getNumberOfLitPixels(newImage)
def do2(enhancement,image):
extendedImage = prepareImage(image, 100)
newImage = imageEnhancement(extendedImage, enhancement, 50)
return getNumberOfLitPixels(newImage)
def getNumberOfLitPixels(image):
litPixels = 0
for line in image:
litPixels += len([x for x in line if x == '#'])
return litPixels
def imageEnhancement(image, enhancement, repetitions):
for _ in range(repetitions):
image = enhanceImage(image, enhancement)
newImage = []
for lineNumber,line in enumerate(image):
if lineNumber == 0 or lineNumber == len(image) - 1:
continue
newImage.append(line[1:-1])
image = deepcopy(newImage)
return image
def enhanceImage(image, enhancement):
newImage = deepcopy(image)
for lineNumber,line in enumerate(image):
if lineNumber == 0 or lineNumber == len(image) - 1:
continue
for position,_ in enumerate(line):
if position == 0 or position == len(line) - 1:
continue
neighbors = getNeighbors(image, lineNumber, position)
pixels = ''
for neighbor in neighbors:
pixels += str(neighbor)
index = int(pixels, 2)
enhancementPixel = enhancement[index]
newImage[lineNumber][position] = enhancementPixel
return newImage
def getNeighbors(image, lineNumber, position):
neighbors = []
for lineDiff in [-1,0,1]:
for posDiff in [-1,0,1]:
neighbors.append(image[lineNumber + lineDiff][position + posDiff])
neighbors = [0 if x == '.' else 1 for x in neighbors]
return neighbors
def prepareImage(image, additionSize):
newImage = []
emptyLine = ['.' for x in range(additionSize)] + ['.' for x in range(len(image[0]))] + ['.' for x in range(additionSize)]
for _ in range(additionSize):
newImage.append(deepcopy(emptyLine))
for line in image:
newImage.append(['.' for x in range(additionSize)] + [x for x in line] + ['.' for x in range(additionSize)])
for _ in range(additionSize):
newImage.append(deepcopy(emptyLine))
return newImage
def do():
strInput = readInputFile(20)
enhancement,image = strInput.split('\n\n')
image = image.split('\n')
print(do1(enhancement,image))
print(do2(enhancement,image))
print('done')
do()<file_sep>/2019/day12.py
import re
input = """<x=14, y=2, z=8>
<x=7, y=4, z=10>
<x=1, y=17, z=16>
<x=-4, y=-1, z=1>"""
def do():
splitInput = input.split('\n')
moons = getMoons(splitInput)
simulate(moons, 1000)
energy = calculateEnergy(moons)
return energy
def do2():
splitInput = input.split('\n')
moons = getMoons(splitInput)
steps = simulateEndless(moons)
return steps
def getMoons(splitInput):
moons = {}
for i in range(len(splitInput)):
moons[i] = {}
moon = splitInput[i]
match = re.match('<x=([-]?[0-9]+), y=([-]?[0-9]+), z=([-]?[0-9]+)>', moon)
positionX, positionY, positionZ = [int(x) for x in match.groups()]
moons[i]['posX'] = positionX
moons[i]['posY'] = positionY
moons[i]['posZ'] = positionZ
moons[i]['velX'] = 0
moons[i]['velY'] = 0
moons[i]['velZ'] = 0
return moons
def simulate(moons, time):
for i in range(time):
for observedMoon in moons.keys():
for otherMoon in moons.keys():
#x
if moons[observedMoon]['posX'] < moons[otherMoon]['posX']:
moons[observedMoon]['velX'] += 1
elif moons[observedMoon]['posX'] > moons[otherMoon]['posX']:
moons[observedMoon]['velX'] -= 1
#y
if moons[observedMoon]['posY'] < moons[otherMoon]['posY']:
moons[observedMoon]['velY'] += 1
elif moons[observedMoon]['posY'] > moons[otherMoon]['posY']:
moons[observedMoon]['velY'] -= 1
#z
if moons[observedMoon]['posZ'] < moons[otherMoon]['posZ']:
moons[observedMoon]['velZ'] += 1
elif moons[observedMoon]['posZ'] > moons[otherMoon]['posZ']:
moons[observedMoon]['velZ'] -= 1
moveMoons(moons)
return moons
def simulateEndless(moons):
steps = 0
while True:
for observedMoon in moons.keys():
for otherMoon in moons.keys():
#x
if moons[observedMoon]['posX'] < moons[otherMoon]['posX']:
moons[observedMoon]['velX'] += 1
elif moons[observedMoon]['posX'] > moons[otherMoon]['posX']:
moons[observedMoon]['velX'] -= 1
#y
if moons[observedMoon]['posY'] < moons[otherMoon]['posY']:
moons[observedMoon]['velY'] += 1
elif moons[observedMoon]['posY'] > moons[otherMoon]['posY']:
moons[observedMoon]['velY'] -= 1
#z
if moons[observedMoon]['posZ'] < moons[otherMoon]['posZ']:
moons[observedMoon]['velZ'] += 1
elif moons[observedMoon]['posZ'] > moons[otherMoon]['posZ']:
moons[observedMoon]['velZ'] -= 1
moveMoons(moons)
steps += 1
if moons[0]['posX'] == 14 and moons[1]['posX'] == 7 and moons[2]['posX'] == 1 and moons[3]['posX'] == -4 and moons[0]['posY'] == 2 and moons[1]['posY'] == 4 and moons[2]['posY'] == 17 and moons[3]['posY'] == -1:
print(steps)
break
return steps
def moveMoons(moons):
for moon in moons.keys():
moons[moon]['posX'] += moons[moon]['velX']
moons[moon]['posY'] += moons[moon]['velY']
moons[moon]['posZ'] += moons[moon]['velZ']
def calculateEnergy(moons):
totalEnergy = 0
for moon in moons.keys():
potentialEnergy = 0
kineticEnergy = 0
potentialEnergy += abs(moons[moon]['posX'])
potentialEnergy += abs(moons[moon]['posY'])
potentialEnergy += abs(moons[moon]['posZ'])
kineticEnergy += abs(moons[moon]['velX'])
kineticEnergy += abs(moons[moon]['velY'])
kineticEnergy += abs(moons[moon]['velZ'])
moons[moon]['energy'] = potentialEnergy * kineticEnergy
totalEnergy += potentialEnergy * kineticEnergy
return totalEnergy
#part1
#print(do())
#part2
print(do2())<file_sep>/2020/day15.py
from HelperFunctions import inputsplit
from HelperFunctions import convertToInt
def do1(puzzleInput):
numbers, lastNumber = prepareInput(puzzleInput)
resultNumber = play(numbers, lastNumber, 2020)
return resultNumber
def do2(puzzleInput):
numbers, lastNumber = prepareInput(puzzleInput)
resultNumber = play(numbers, lastNumber, 30000000)
return resultNumber
def prepareInput(puzzleInput):
intNumbers = convertToInt(puzzleInput)
numbers = {}
for i, number in enumerate(intNumbers):
numbers[number] = [1, [i + 1]]
lastNumber = number
return [numbers, lastNumber]
def play(numbers, lastNumber, maxIterations):
i = 1 + numbers[lastNumber][1][-1]
while (i < maxIterations + 1):
oldLastNumber = lastNumber
if numbers[oldLastNumber][0] == 1:
lastNumber = 0
else:
lastNumber = numbers[oldLastNumber][1][-1] - numbers[oldLastNumber][1][-2]
if lastNumber not in numbers.keys():
numbers[lastNumber] = [0, []]
numbers[lastNumber][0] += 1
numbers[lastNumber][1].append(i)
numbers[lastNumber][1] = numbers[lastNumber][1][-2:]
i += 1
return lastNumber
def do():
with open ('Input/day15.txt') as f:
strInput = f.read()
splitInput = inputsplit(strInput, ',')
print(do1(splitInput))
print(do2(splitInput))
do()<file_sep>/2021/day9.py
from types import CodeType
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
def do1(splitInput):
map, _ = generateMap(splitInput)
lowPoints = []
for lineNumber,line in enumerate(map):
for position,height in enumerate(line):
if height == 9:
continue
if line[position - 1] <= height:
continue
if line[position + 1] <= height:
continue
if map[lineNumber - 1][position] <= height:
continue
if map[lineNumber + 1][position] <= height:
continue
lowPoints.append(height)
riskLevels = [x + 1 for x in lowPoints]
return sum(riskLevels)
def do2(splitInput):
map,basins = generateMap(splitInput)
basinNumber = 0
for lineNumber,line in enumerate(map):
for position,height in enumerate(line):
if height == 9:
continue
if basins[lineNumber][position] != -1:
continue
search(lineNumber, position, map, basins, basinNumber)
basinNumber += 1
count = {}
for i in range(basinNumber):
sumI = 0
for line in basins:
sumI += len([x for x in line if x == i])
count[i] = sumI
maxBassins = [x for x in sorted(count.values(), reverse=True)][0:3]
return maxBassins[0] * maxBassins[1] * maxBassins[2]
def generateMap(splitInput):
map = []
basins = []
map.append([9] + [9 for x in splitInput[0]] + [9])
basins.append([-1] + [-1 for x in splitInput[0]] + [-1])
for line in splitInput:
bassinLine = [-1] + [-1 for x in line] + [-1]
intLine = [9] + convertToInt(line) + [9]
map.append(intLine)
basins.append(bassinLine)
map.append([9] + [9 for x in splitInput[0]] + [9])
basins.append([-1] + [-1 for x in splitInput[0]] + [-1])
return (map,basins)
def search(line, position, map, basins, bassinNumber):
currentHeight = map[line][position]
while currentHeight != 9:
if basins[line][position] != -1 and basins[line][position] != bassinNumber:
print('Something is wrong')
return
if basins[line][position] == bassinNumber:
return
basins[line][position] = bassinNumber
search(line - 1, position, map, basins, bassinNumber)
search(line + 1, position, map, basins, bassinNumber)
search(line, position - 1, map, basins, bassinNumber)
search(line, position + 1, map, basins, bassinNumber)
def do():
strInput = readInputFile(9)
splitInput = strInput.split('\n')
print(do1(splitInput))
print(do2(splitInput))
print('done')
do()<file_sep>/2022/day17.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
rocks = {0:[(0,0),(1,0),(2,0),(3,0)], 1:[(1,0),(0,1),(1,1),(2,1),(1,2)], 2:[(0,0),(1,0),(2,0),(2,1),(2,2)], 3:[(0,0),(0,1),(0,2),(0,3)], 4:[(0,0),(0,1),(1,0),(1,1)]}
def do1(movement):
rockPositions = set([(0,0),(1,0),(2,0),(3,0),(4,0),(5,0),(6,0)])
position = 0
floor = 0
fallenRocks = 0
while fallenRocks < 2022:
rock = rocks[fallenRocks % 5]
leftCorner = (2,floor + 4)
falling = True
while falling:
leftCorner = getPushed(movement[position], rockPositions, leftCorner, rock)
position = (position + 1) % len(movement)
falling,leftCorner = fallDown(leftCorner, rockPositions, rock)
fallenRocks += 1
leftCornerX,leftCornerY = leftCorner
for rockPartX,rockPartY in rock:
rockPositions.add((leftCornerX + rockPartX, leftCornerY + rockPartY))
floor = max(floor, leftCornerY + rockPartY)
return max([y for (x,y) in rockPositions])
def getPushed(movement, rockPositions, leftCorner, rock):
leftCornerX,leftCornerY = leftCorner
match movement:
case '<':
leftCornerX -= 1
for rockPartX,rockPartY in rock:
if (leftCornerX + rockPartX,leftCornerY + rockPartY) in rockPositions or leftCornerX + rockPartX < 0:
return leftCorner
return (leftCornerX,leftCornerY)
case '>':
leftCornerX += 1
for rockPartX,rockPartY in rock:
if (leftCornerX + rockPartX,leftCornerY + rockPartY) in rockPositions or leftCornerX + rockPartX > 6:
return leftCorner
return (leftCornerX,leftCornerY)
def fallDown(leftCorner, rockPositions, rock):
leftCornerX,leftCornerY = leftCorner
leftCornerY -= 1
for rockPartX,rockPartY in rock:
if (leftCornerX + rockPartX,leftCornerY + rockPartY) in rockPositions:
return False,leftCorner
return True,(leftCornerX,leftCornerY)
def do2(movement):
#cycle starts at position = 9 with 1720 fallen rocks and floor height = 2691, floor difference from cycle to cycle is +2728 with 1725 new rocks
#missing rocks to reach 1000000000000 are 1605 which have a height of 2524
return 2691 + (int((1000000000000-1720) / 1725)) * 2728 + 2524
def do():
strInput = readInputFile(17)
print(do1(strInput))
print(do2(strInput))
print('done')
do()<file_sep>/2020/day12.py
from HelperFunctions import inputsplit
from enum import Enum
def do1(puzzleInput):
east = 0
north = 0
direction = 0
for movement in puzzleInput:
action = movement[0]
count = int(movement[1:])
east, north, direction = move(east, north, action, count, direction)
return abs(north) + abs(east)
def do2(puzzleInput):
wayNorth = 1
wayEast = 10
east = 0
north = 0
for movement in puzzleInput:
action = movement[0]
count = int(movement[1:])
wayNorth, wayEast, east, north = moveWithWaypoint(wayNorth, wayEast, east, north, action, count)
return abs(north) + abs(east)
def moveWithWaypoint(wayNorth, wayEast, east, north, action, count):
if action == 'N':
wayNorth += count
elif action == 'S':
wayNorth -= count
elif action == 'E':
wayEast += count
elif action == 'W':
wayEast -= count
elif action == 'L':
wayNorth, wayEast = turnWaypoint(wayNorth, wayEast, 'L', count)
elif action == 'R':
wayNorth, wayEast = turnWaypoint(wayNorth, wayEast, 'R', count)
elif action == 'F':
east, north = driveToWaypoint(east, north, wayNorth, wayEast, count)
return [wayNorth, wayEast, east, north]
def turnWaypoint(wayNorth, wayEast, turnOrder, count):
if turnOrder == 'R':
if count == 90:
wayNorthtmp = wayNorth
wayNorth = -wayEast
wayEast = wayNorthtmp
elif count == 180:
wayNorth = -wayNorth
wayEast = -wayEast
elif count == 270:
wayNorthtmp = wayNorth
wayNorth = wayEast
wayEast = -wayNorthtmp
elif turnOrder == 'L':
return turnWaypoint(wayNorth, wayEast, 'R', 360 - count)
return [wayNorth, wayEast]
def driveToWaypoint(east, north, wayNorth, wayEast, count):
for _ in range(count):
east, north,_ = move(east, north, 'N', wayNorth)
east, north,_ = move(east, north, 'E', wayEast)
return [east, north]
def move(east, north, action, count, direction=0):
if action == 'N':
north += count
elif action == 'S':
north -= count
elif action == 'E':
east += count
elif action == 'W':
east -= count
elif action == 'L':
direction = turn(direction, 'L', count)
elif action == 'R':
direction = turn(direction, 'R', count)
elif action == 'F':
east, north = driveForward(east, north, direction, count)
else:
print('error')
return [east, north, direction]
def turn(direction, turnOrder, degrees):
if turnOrder == 'R':
direction = (direction + int(degrees / 90)) % 4
elif turnOrder == 'L':
direction = (direction + (4 - int(degrees / 90))) % 4
return direction
def driveForward(east, north, direction, count):
actions = {0: 'E', 1: 'S', 2: 'W', 3: 'N'}
action = actions.get(direction, 'X')
east, north, _ = move(east, north, action, count, direction)
return [east, north]
def do():
with open ('Input/day12.txt') as f:
strInput = f.read()
splitInput = inputsplit(strInput, '\n')
print(do1(splitInput))
print(do2(splitInput))
do()<file_sep>/2022/day9.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
TPositions = set()
tailPositions = set()
def do1(splitInput):
HPos = (0,0)
TPos = (0,0)
TPositions.add(TPos)
for line in splitInput:
command,steps = line.split(' ')
for _ in range(int(steps)):
match command:
case 'R':
HPos = (HPos[0] + 1, HPos[1])
case 'L':
HPos = (HPos[0] - 1, HPos[1])
case 'D':
HPos = (HPos[0], HPos[1] - 1)
case 'U':
HPos = (HPos[0], HPos[1] + 1)
TPos = updatePos(HPos, TPos)
TPositions.add(TPos)
return len(TPositions)
def do2(splitInput):
positions = [(0,0) for x in range(10)]
tailPositions.add(positions[-1])
for line in splitInput:
command,steps = line.split(' ')
for _ in range(int(steps)):
headPos = positions[0]
match command:
case 'R':
positions[0] = (headPos[0] + 1, headPos[1])
case 'L':
positions[0] = (headPos[0] - 1, headPos[1])
case 'D':
positions[0] = (headPos[0], headPos[1] - 1)
case 'U':
positions[0] = (headPos[0], headPos[1] + 1)
for index,knot in enumerate(positions[1:]):
positions[index + 1] = updatePos(positions[index], knot)
tailPositions.add(positions[-1])
return len(tailPositions)
def updatePos(HPos, TPos):
TPositions.add(TPos)
#same row or colum
if TPos[0] == HPos[0]:
match HPos[1] - TPos[1]:
case 2:
TPos = (TPos[0], TPos[1] + 1)
case -2:
TPos = (TPos[0], TPos[1] - 1)
elif TPos[1] == HPos[1]:
match HPos[0] - TPos[0]:
case 2:
TPos = (TPos[0] + 1, TPos[1])
case -2:
TPos = (TPos[0] - 1, TPos[1])
#diagonally
elif abs(HPos[0] - TPos[0]) + abs(HPos[1] - TPos[1]) == 2:
return TPos
else:
# H is right from T:
if HPos[0] > TPos[0]:
if HPos[1] > TPos[1]:
TPos = (TPos[0] + 1, TPos[1] + 1)
else:
TPos = (TPos[0] + 1, TPos[1] - 1)
else:
if HPos[1] > TPos[1]:
TPos = (TPos[0] - 1, TPos[1] + 1)
else:
TPos = (TPos[0] - 1, TPos[1] - 1)
return TPos
def do():
strInput = readInputFile(9)
splitInput = strInput.split('\n')
print(do1(splitInput))
print(do2(splitInput))
print('done')
do()<file_sep>/2020/day10.py
from HelperFunctions import inputsplit
from HelperFunctions import convertToInt
def do1(puzzleInput):
prepareInput(puzzleInput)
differences = getDifferences(puzzleInput)
return (differences.count(1)) * (differences.count(3))
def do2(puzzleInput):
prepareInput(puzzleInput)
return getNumberOfPossibilities(puzzleInput)
def prepareInput(puzzleInput):
puzzleInput.insert(0,0)
puzzleInput.insert(-1, max(puzzleInput) + 3)
puzzleInput.sort()
def getDifferences(puzzleInput):
differences = [puzzleInput[x+1] - puzzleInput[x] for x in range(len(puzzleInput)-1)]
return differences
def getNumberOfPossibilities(puzzleInput):
puzzleInput.reverse()
possibilites = {puzzleInput[0]:1}
for value in puzzleInput[1:]:
sum = 0
for i in [1,2,3]:
sum += possibilites.get(value + i, 0)
possibilites[value] = sum
return possibilites[puzzleInput[-1]]
def do():
with open ('Input/day10.txt') as f:
strInput = f.read()
splitInput = inputsplit(strInput, '\n')
intInput = convertToInt(splitInput)
print(do1(intInput))
print(do2(intInput))
do()<file_sep>/2022/day15.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
import re
def do1(splitInput):
pairs,sensors,beacons = extractSensorsAndBeacons(splitInput)
impossiblePoints = set()
y = 2000000
for sensor,beacon in pairs.items():
sensorX,sensorY = sensor
distance = manhattanDistance(sensor,beacon)
impossible = [(x,y) for x in range(sensorX-distance, sensorX+distance+1) if manhattanDistance(sensor,(x,y)) <= distance]
impossiblePoints = impossiblePoints.union(set(impossible))
sumImpossible = len([x for x in impossiblePoints if x[1] == y])
sumBeacons = len([x for x in beacons if x[1] == y])
sumSensors = len([x for x in sensors if x[1] == y])
return sumImpossible - sumBeacons - sumSensors
def do2(splitInput):
pairs,sensors,beacons = extractSensorsAndBeacons(splitInput)
distances = {}
for sensor,beacon in pairs.items():
distances[sensor] = manhattanDistance(sensor,beacon)
for sensor in sensors:
sensorX,sensorY = sensor
possiblePoints = []
distance = distances[sensor]
x = distance + 1
y = 0
while -distance - 1 <= x <= distance + 1:
possiblePoints.append((sensorX + x, sensorY + y))
x -= 1
if x < 0:
y -= 1
else:
y += 1
for point in possiblePoints:
pointX,pointY = point
if not 0 <= pointX <= 4000000 or not 0 <= pointY <= 4000000:
continue
possible = True
for otherSensor in sensors:
if otherSensor == sensor:
continue
if manhattanDistance(point,otherSensor) <= distances[otherSensor]:
possible = False
break
if possible:
return point[0] * 4000000 + point[1]
def manhattanDistance(a,b):
ax,ay = a
bx,by = b
return abs(ax-bx) + abs(ay-by)
def extractSensorsAndBeacons(splitInput):
pairs = {}
sensors = set()
beacons = set()
for line in splitInput:
match = re.match('Sensor at x=(?P<xSensor>[-]?\d+), y=(?P<ySensor>[-]?\d+): closest beacon is at x=(?P<xBeacon>[-]?\d+), y=(?P<yBeacon>[-]?\d+)', line)
sensorPos = (int(match.group('xSensor')), int(match.group('ySensor')))
beaconPos = (int(match.group('xBeacon')), int(match.group('yBeacon')))
pairs[sensorPos] = beaconPos
sensors.add(sensorPos)
beacons.add(beaconPos)
return pairs,sensors,beacons
def do():
strInput = readInputFile(15)
splitInput = strInput.split('\n')
print(do1(splitInput))
print(do2(splitInput))
print('done')
do()<file_sep>/2022/day2.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
points = {'X':1, 'Y':2, 'Z':3}
choices = ['Y', 'A', 'Z', 'B', 'X', 'C', 'Y']
sameChoice = {'A':'X', 'B':'Y', 'C':'Z'}
def do1(splitInput):
score = 0
for line in splitInput:
opponent,me = line.split(' ')
if [opponent,me] in choices:
winScore = 0
elif [me,opponent] in choices:
winScore = 6
else:
winScore = 3
roundScore = points[me] + winScore
score += roundScore
return score
def do2(splitInput):
score = 0
for line in splitInput:
opponent,result = line.split(' ')
opponentIndex = choices.index(opponent)
match result:
case 'X':
me = choices[opponentIndex + 1]
winScore = 0
case 'Y':
me = sameChoice[opponent]
winScore = 3
case 'Z':
me = choices[opponentIndex - 1]
winScore = 6
roundScore = points[me] + winScore
score += roundScore
return score
def do():
strInput = readInputFile(2)
splitInput = strInput.split('\n')
print(do1(splitInput))
print(do2(splitInput))
print('done')
do()<file_sep>/2021/day21.py
from functools import cache
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
from collections import Counter, deque
die = deque([i for i in range(1,101)])
def do1(player1,player2):
score1,score2,diceRolls = play1(player1, player2)
losingScore = min([score1, score2])
return diceRolls * losingScore
def do2(player1,player2):
wins = play2(player1, player2, 0, 0)
return max(wins)
def getOptions():
options = []
for i in range(1,4):
for j in range(1,4):
for k in range(1,4):
options.append(i+j+k)
counts = Counter()
for option in options:
counts[option] += 1
return counts
def play1(first, second):
score1 = 0
score2 = 0
diceRolls = 0
while score1 < 1000 and score2 < 1000:
diceNumber = getDice()
diceRolls += 3
first = (first - 1 + diceNumber) % 10 + 1
score1 += first
if score1 >= 1000:
return score1,score2,diceRolls
diceNumber = getDice()
diceRolls += 3
second = (second - 1 + diceNumber) % 10 + 1
score2 += second
if score2 >= 1000:
return score1,score2,diceRolls
return score1,score2,diceRolls
@cache
def play2(first, second, score1, score2):
if score1 >= 21:
return (1,0)
elif score2 >= 21:
return (0,1)
else:
counts = getOptions()
result = (0,0)
for option,number in counts.items():
newfirst = (first - 1 + option) % 10 + 1
newscore1 = score1 + newfirst
if newscore1 < 21:
counts2 = getOptions()
for option2,number2 in counts2.items():
newsecond = (second - 1 + option2) % 10 + 1
newscore2 = score2 + newsecond
result1, result2 = play2(newfirst,newsecond,newscore1,newscore2)
result = (result[0] + number * number2 * result1, result[1] + number * number2 * result2)
else:
result = (result[0] + number, result[1])
return result
def getDice():
diceNumbers = []
for _ in range(3):
diceNumbers.append(die[0])
die.rotate(-1)
return sum(diceNumbers)
def do():
strInput = readInputFile(21)
player1,player2 = convertToInt(strInput.split('\n'))
print(do1(player1,player2))
print(do2(player1,player2))
print('done')
do()<file_sep>/2020/HelperFunctions.py
def inputsplit(input, separator=' '):
return input.split(separator)
def convertToInt(input):
numbers = []
for stringNumber in input:
numbers.append(int(stringNumber))
return numbers<file_sep>/2022/day4.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
def do1(splitInput):
fullyContained = 0
for pair in splitInput:
elf1Assignment,elf2Assignment = getElvesAssignments(pair)
if elf1Assignment.issubset(elf2Assignment) or elf2Assignment.issubset(elf1Assignment):
fullyContained += 1
return fullyContained
def do2(splitInput):
overlaps = 0
for pair in splitInput:
elf1Assignment,elf2Assignment = getElvesAssignments(pair)
if elf1Assignment.intersection(elf2Assignment):
overlaps += 1
return overlaps
def getElvesAssignments(pair):
assignments = []
for elf in pair.split(','):
elfStart,elfStop = [int(x) for x in elf.split('-')]
elfAssignment = set([x for x in range(elfStart, elfStop + 1)])
assignments.append(elfAssignment)
return assignments
def do():
strInput = readInputFile(4)
splitInput = strInput.split('\n')
print(do1(splitInput))
print(do2(splitInput))
print('done')
do()<file_sep>/2021/day6.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
from copy import deepcopy
from collections import defaultdict
def do1(splitInput):
fishes = growFishes(splitInput, 80)
sumOfFishes = sum([x for x in fishes.values()])
return sumOfFishes
def do2(splitInput):
fishes = growFishes(splitInput, 256)
sumOfFishes = sum([x for x in fishes.values()])
return sumOfFishes
def growFishes(splitInput, days):
fishes = defaultdict(lambda: 0)
for fish in splitInput:
fishes[fish] += 1
for i in range(days):
newFishes = defaultdict(lambda: 0)
for key,value in fishes.items():
if key == 0:
newFishes[8] += value
newFishes[6] += value
else:
newFishes[key - 1] += value
fishes = deepcopy(newFishes)
return fishes
def do():
strInput = readInputFile(6)
splitInput = strInput.split(',')
intInput = convertToInt(splitInput)
print(do1(intInput))
print(do2(intInput))
print('done')
do()<file_sep>/2019/day16.py
input = """59767332893712499303507927392492799842280949032647447943708128134759829623432979665638627748828769901459920331809324277257783559980682773005090812015194705678044494427656694450683470894204458322512685463108677297931475224644120088044241514984501801055776621459006306355191173838028818541852472766531691447716699929369254367590657434009446852446382913299030985023252085192396763168288943696868044543275244584834495762182333696287306000879305760028716584659188511036134905935090284404044065551054821920696749822628998776535580685208350672371545812292776910208462128008216282210434666822690603370151291219895209312686939242854295497457769408869210686246"""
def inputSplit(input):
intInput = []
for x in input:
intInput.append(int(x))
return intInput
def do():
splitInput = inputSplit(input)
result = splitInput.copy()
for i in range(100):
for outputPos in range(len(splitInput)):
pattern = createPattern(outputPos)
result[outputPos] = applyPattern(pattern, splitInput)
splitInput = result.copy()
return result
def applyPattern(pattern, splitInput):
patternPos = 1
sum = 0
for pos in range(len(splitInput)):
sum += splitInput[pos] * pattern[patternPos]
patternPos = (patternPos + 1) % len(pattern)
return abs(sum) % 10
def createPattern(pos):
pattern = [0, 1, 0, -1]
resultPattern = []
for position in range(len(pattern)):
for repetition in range(pos + 1):
resultPattern.append(pattern[position])
return resultPattern
print(do()[:8])<file_sep>/2018/day2.py
input = """<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
ln<KEY>
lt<KEY>
ln<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
ln<KEY>
ln<KEY>
ln<KEY>
<KEY>
<KEY>
wn<KEY>
ln<KEY>
ln<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
ln<KEY>
ln<KEY>
ln<KEY>
fn<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
<KEY>
ln<KEY>
ln<KEY>
ln<KEY>
ln<KEY>
en<KEY>
gn<KEY>
ln<KEY>
ln<KEY>
ln<KEY>
ln<KEY>
tn<KEY>
ln<KEY>
ln<KEY>
ln<KEY>
l<KEY>
ln<KEY>
ln<KEY>
ln<KEY>
ln<KEY>
ln<KEY>
ln<KEY>
ln<KEY>
ln<KEY>
ln<KEY>
l<KEY>
ln<KEY>"""
def inputsplit(input):
return input.split()
def doPartOne():
splitInput = inputsplit(input)
twos = 0
threes = 0
for thing in splitInput:
two = True
three = True
for letter in thing:
if thing.count(letter) == 2 and two:
twos += 1
two = False
if thing.count(letter) == 3 and three:
threes += 1
three = False
thing = thing.replace(letter, '')
print(str(twos) + ' ' + str(threes) + ' ' + str(twos*threes))
def doPartTwo():
position = 0
splitInput = inputsplit(input)
while(position < len(splitInput[0])):
newInput = []
for thing in splitInput:
thing = thing[:position] + thing[(position + 1):]
newInput.append(thing)
for newthing in newInput:
if newInput.count(newthing) == 2:
print(newthing)
return
position += 1
doPartOne()
doPartTwo()<file_sep>/2019/day6.py
input = """6CF)4J7
RC4)H87
LMS)RL4
KGS)1QW
H8N)KW5
JJM)YPS
P1W)ZKT
BSY)FMR
9T5)JK4
2X2)NQ5
H6R)FLY
8N9)H7L
5LR)TYT
VM7)V5V
GVF)Q8P
PQV)9NB
4W3)V84
H59)B3G
RGW)82C
BTQ)VRF
KQW)TNC
RT3)JHN
ZLY)15X
YJJ)55L
8Q2)6S7
963)PNL
8F3)WPH
H2Z)LMN
MFK)DMJ
JGN)H4X
P1C)Y9K
92D)8YS
2M2)PQ1
YY6)PRG
3J8)LBX
2WD)TK3
CNS)BJL
B53)JJ5
NND)CBM
FHS)RP5
RMS)6VH
FBB)QVC
SD2)WPN
BXL)76Q
V8H)LCW
MXC)2W2
CCX)KC9
JSQ)2T8
7HM)D24
XN9)N36
J3X)PRZ
6VQ)8ZV
QR4)MTL
KVT)V47
PXR)VFJ
VZN)P7W
WK6)72B
R46)J6N
5M4)LZB
4PL)V6R
WWR)BJ1
TPX)NRM
BS7)X7M
JJ5)8N2
V9N)D6W
7K2)2LZ
3JZ)FV1
2DJ)6N1
RC5)L5D
2RG)FK8
V8M)CL6
KLY)MBD
DMJ)KVD
6M6)6WX
HB1)1HD
KJW)G61
HZF)B1Q
2N4)73R
H8G)8XL
ZGK)RSY
35Y)FSR
H34)GLB
9MV)WR9
NBS)RV6
6QM)KLM
HN3)K2T
LCB)GNZ
LDJ)D3T
5PC)512
BXH)6MZ
XS7)W5B
LF3)6LJ
3Z8)ZTJ
3NT)9D9
65F)JVH
V6V)C9B
KTQ)KG8
6WP)92D
X4M)WGG
66R)B53
B5C)2MP
FC4)6T3
WWR)VRD
9DG)P94
XFV)TQQ
4LK)V21
2H8)HVS
JHN)QHZ
2GV)VSL
581)XRN
THC)ZGK
CB8)R2T
FN9)4Y9
1KH)RHQ
YF2)FNW
ZBW)JLW
YCK)R9D
2SH)X2M
512)W7P
TDK)FN9
TH8)HQZ
JK4)P62
BLG)CWW
V84)VYC
BTY)NXZ
8GC)DX1
CN5)YPZ
Y6J)CQP
L1X)NXF
SQ7)3QL
8KD)PS4
1SW)RHW
7X4)S86
RGS)581
2BC)DVS
6J8)XXQ
Q3S)TKF
T2K)TRL
NY4)16L
96Z)NMX
J1W)YRJ
8C3)29M
VRF)VX2
LD6)Y6M
RHX)FN3
J6N)LMY
BSB)JXV
PN4)L1X
M92)JPT
TBX)324
8RN)QKN
GNZ)J2D
GZ1)WJ4
783)96Z
SLD)L3C
9FK)V9L
D3T)PM2
1JL)MKH
CKL)PYR
SZD)YF2
8LD)4GQ
MQ2)JCP
CTW)GZ1
M7W)8NR
FWH)GBQ
1CX)BTC
YJL)RGX
FLY)ZDD
NCJ)8GC
PM2)2YG
T82)KM8
4M3)Q71
LGQ)JYC
K4Y)287
J48)THC
CNV)NCJ
9P7)SZY
QXP)XM5
S5Z)BSY
59Q)SLL
S79)16C
W66)JL4
L6Q)CZ2
7CB)7HP
DQ4)CLP
PQ1)FCR
5QP)163
RJB)H3J
CH5)25F
TQG)VLN
SDT)J67
1VL)X2B
5SM)G71
RSY)1J4
CZP)XNT
57T)37Z
1J4)3B2
7X4)WNS
BNT)NND
QMS)DVH
VW6)6QM
VFJ)4XH
17T)NLM
N8T)7HM
QPS)7LM
XXQ)2KV
WBS)8RF
95F)3Z8
NR1)WQM
S75)WNT
ZJ5)7WS
YNF)RDQ
2DY)XBV
7LM)V48
C78)64H
VHR)L2P
V47)9QN
9C5)Y5S
9D9)QDT
7DC)2DJ
H8P)H6P
QH4)X4N
K19)CYL
CXY)CMV
G8D)FDN
4Z3)LVF
V1Z)YHN
GM3)B2Q
LVF)VZH
2LZ)YOU
FNW)1R5
36J)2QT
2Y4)69H
LTZ)JGN
GTN)MZ3
FQX)WZP
3M8)P7L
16L)H78
9B1)NTB
PB7)86H
Z3P)T9T
M1X)KK5
6PS)5FQ
55L)2WZ
174)6GC
86H)2W7
WXH)R15
3B2)3Q6
BGQ)SPZ
1QW)NJT
WCK)5R8
ZKT)MTH
Z7S)67J
FK8)5J4
WJM)H83
4Y9)Z9B
LKC)3MW
6FM)MC3
163)68L
XJS)2PD
NVB)C8Q
WTV)S32
V9L)SVG
5HW)7LG
BTC)DX6
TKF)2DX
JJK)NPF
LQN)DSV
21B)JTT
9H8)ZY4
72B)W1Y
MF8)M3Q
6WF)SQ3
N34)4HL
1KB)32T
VYP)H6F
TTC)XHP
QM4)H2Z
NRM)7FK
ZGK)V13
XRN)361
324)ZMX
1PY)PH7
PDS)VLX
M88)XD4
1DZ)VF8
YL2)J9J
6GB)NR1
VX2)JJM
X4N)FN1
KK5)MZX
7ZT)GLZ
JDG)GGM
Q4N)8RN
JYC)N5Z
MTL)SPD
1KQ)135
9JX)FHS
NSF)PYT
QWF)QX9
FY1)48P
V4W)GXX
L5D)8KQ
P1C)F46
5R8)M1X
NSM)SV5
366)CXY
D24)Q83
ZKG)SD2
QKN)MC4
P4P)RK6
9QN)K4Y
38D)TJH
RG6)SZD
JL4)GQQ
Y6M)3NT
FR7)C3Q
DNH)7YW
SDL)M5X
JFT)YY6
5D9)KVT
JBL)GM3
C4V)FYC
KW5)Y7D
V76)VSH
CMV)ZKY
TK3)5KN
KDS)X34
NMY)QYZ
MDP)GVL
QSS)CY8
QFX)ZN1
Q54)VJP
VSH)3DK
ZY4)B19
X6Y)15G
L3C)78D
LDV)SJT
8M7)6VQ
22Z)BTQ
RHQ)NFK
9NT)DQ4
C9B)WB9
HGD)QXP
YDX)YMZ
VMQ)8C3
9RM)QTY
GQQ)9C1
LSG)KJW
6MS)GVF
ZTL)LLK
S86)W19
1FV)LGQ
C8Q)P4P
DMP)Y1X
48P)LGX
MZ3)9B1
LKH)2WL
NZJ)TNJ
F67)L34
9Y3)783
ZNJ)6WF
WX6)ZSC
15G)RTW
QHP)9YN
DQH)KY6
4LF)LY8
XHR)GK1
3MW)SRY
Q8N)RPX
R9X)SZL
V85)FR7
MVW)QLB
1DY)H29
43D)NY4
MKH)SBX
5FQ)ZT8
P97)2JM
DSV)RGW
37Z)5SJ
3WG)KTQ
Z9G)2N4
K7Q)HQV
BX1)2H8
7WS)G27
WHN)2DL
YHN)9YB
1JN)BGQ
PYP)VQ8
VXC)917
13Z)XTZ
9WT)N8Q
VVH)MP7
WDM)RC5
G8T)WHN
VYC)MR6
XM5)FWH
N8Q)V77
2YM)HH9
L4J)8G9
1TG)S6H
XCC)TD8
KRF)4KZ
19N)9FK
SMS)V1K
8N2)MZS
869)8F8
JGD)PYW
76V)CLW
NDZ)VM7
VLX)6WD
895)38D
1XZ)Q34
VF8)1F6
TNC)7DC
16C)MWQ
LDJ)C4L
ZV6)7XJ
NXZ)H34
BLS)4MM
GG6)NKG
BCD)KT9
PYW)LSG
LNP)7XQ
MZX)BY9
RGX)J8P
S32)DSS
J9J)LTZ
39P)S75
CNS)PDZ
PYW)CHG
Z19)V8Q
68L)9WR
V6R)MVW
LLK)MYP
9MV)MQT
1FH)4CK
P4N)MMM
4TD)96J
V77)CH4
MMW)CTW
MLN)3CS
W8G)2JS
4KZ)HBT
5PM)6PP
C81)JSQ
V11)WK6
FCR)MVH
YR2)KLY
W1H)XTX
SKL)NQG
2YM)VL7
M8K)6HN
1K6)JX8
ZDD)H1W
4JW)NV8
GS2)CMP
RDM)7XB
3QB)GTN
D6W)MJT
NRK)6PS
7WS)B1L
PKH)L4J
G61)WTV
2T8)8Q2
TL6)W7Z
1TC)KXM
1VL)DZD
LVZ)1DZ
1TQ)S3R
7BY)ZB6
JPT)1JN
LF3)K91
37D)LKM
9LN)VZN
MQT)Q1F
M6X)GTS
CNH)1WS
6GC)BSM
1F6)88V
FKP)XQZ
QX9)5SM
1HD)VP7
MMQ)RT3
QXX)9P7
GLT)SQ7
HQP)TLR
K2T)P6X
TTZ)Z7S
D49)3NV
DFD)V29
LPW)877
ZKT)BXL
YHN)TKD
FV1)9Y3
3ZB)57Z
WN1)35Y
SQ3)ZCF
DJF)ZNJ
R61)LCB
BMX)YFT
W5B)3VM
46C)174
BJ1)2XP
XZJ)KN9
2D7)N8T
TTF)KDS
1T5)8M7
Y5Q)SKL
6C8)WHW
QBH)6PZ
6RD)7BY
Z2Y)1KB
J3Q)X6Y
PBR)2D7
JLW)2CY
RP5)54P
PYT)9HC
N5M)FY1
MWG)N2M
V1K)M7W
55L)869
M3Q)Q8N
FSR)ZV6
MX8)RQF
LKM)SAN
KKP)WDM
FN1)1DW
WB9)VDR
G98)RMS
H7L)CCN
TL3)LJF
YNZ)K8V
KL2)66B
M28)P9Z
Q34)Y28
TNJ)NSM
W25)R9X
8XL)GS2
ZB6)26Z
BYR)5QP
MVH)BNW
TKD)P1C
9JW)WX6
8LC)J3Q
H8F)Y14
347)7SJ
8VL)RC4
38T)1FV
9FM)M39
CH4)LVQ
LNV)QPS
H87)FPL
9YN)LKH
9HB)PBR
9WR)NWL
X7M)BYR
3GM)N7X
67J)RJB
2XV)LNV
3VX)WWR
1R5)VMD
9HC)BTY
GVL)CVB
D8J)5LR
M39)VW6
NPF)MFK
7NQ)6M9
3DK)P76
ZQG)J1W
JCP)TBX
DX1)9DG
917)LDV
CBT)M9J
G71)H8P
4J7)FZJ
RGX)CB8
63W)P1W
V8Q)2DY
H42)YGG
RY7)1V6
W7Z)CYN
BGL)JJ8
TLR)X2S
X4H)2SB
MC4)QR4
Q8P)CCX
P8R)43D
J3R)VS6
TPK)9H8
D17)NMY
G27)9S9
1SV)RWF
MQ2)B47
L2P)VMF
V13)Y5Q
L5B)YJJ
WNT)MZF
B47)WN1
KN9)9VX
LFJ)GPB
F82)855
BD5)MLN
SBX)CBT
7XQ)H65
VJP)6WB
PH7)WKG
LBF)1SW
2YG)FSB
7LM)8DN
913)5D9
VLN)BLG
FM6)5PM
5W2)7W8
BY9)6M6
ZN1)FP5
2WZ)BSB
D24)4LK
8NR)R8W
414)QSS
ZMX)4TD
3VM)ZLY
HT3)LDJ
XDR)CJ6
R2T)22Z
L34)RY7
7FK)17T
X2S)TTZ
714)9C5
BNR)GYJ
RQF)KQW
3F9)GWN
FL6)DNH
6WB)YL4
7MT)V1Z
Q1F)LBY
PS4)Q54
82C)MDP
77H)MGJ
CVB)DXB
HGD)FBB
ZTJ)XH9
FXS)JP8
KB9)WYZ
C4L)XG8
C3Q)YR2
96J)F8M
W53)6C8
LZB)L6Q
Z78)62Y
361)G8D
FY1)RDM
S5Z)V9N
23W)PN4
SRX)Z3P
PRZ)PXR
7NF)R58
QTY)WY4
897)V3S
GVF)ZV3
PTP)21B
37G)H59
Y3R)LVZ
CWH)C81
W9M)GXC
V29)CKL
NXR)91Q
Y84)6RD
G2K)2JD
MR6)1CX
QDT)F67
ZM3)1BY
XHP)H8F
WVL)9JX
NRM)Y4R
CY8)CHJ
H6P)XJS
96Z)MT8
P6X)4M3
XQZ)SC7
J67)3J8
GCR)V85
H65)ZM3
K4Y)W74
WPN)6NJ
F46)LG7
H78)NXR
LBY)G98
FZJ)63T
21B)CT7
7G9)414
2D8)G7Z
QLB)VTR
1V6)VGH
R15)LQP
2QT)Y3R
Q83)7YN
BXL)NV4
J8P)JDG
76Q)C4V
SZY)PTP
7SJ)7G9
C8R)H42
VW6)8LC
135)6MB
4XH)WJM
8KT)93S
G7Z)7NQ
9C1)GSW
TC8)J26
3F4)W8G
CZ2)RPJ
9WT)BCD
LY8)533
548)PKH
K2M)6MQ
2X1)HN1
LSY)R9G
PVN)X3T
H3J)L65
7XB)DT6
DVS)XN9
SGN)DS4
NYD)WHP
DWD)C3V
LM8)MGP
ZCF)VSC
PXN)ZPT
7ZT)CWH
DGH)7K2
VSL)4CC
FP5)Z9G
6F2)MWG
RV6)MNB
8TQ)GTB
YPZ)QL2
14G)SMS
VML)XHR
Y4R)D46
MNB)1K6
FNP)9H6
X1T)5JP
1DW)28V
76P)4MK
WW4)53M
WZP)B5C
DS4)1PZ
B1L)DQW
RHX)RGS
42C)CBV
HQV)9T5
YDV)DFD
KG8)Q7R
72W)BSZ
369)ZBW
9YB)77H
MD3)YT8
YGG)VYP
3QL)289
X2B)548
Y5S)R64
9MM)P8R
W19)382
RPJ)QQG
P9Z)BNT
DNB)CCP
6HN)DMP
MW5)SLD
WRS)HWD
ZKR)186
WQM)LQN
NL4)JBS
WHW)TTC
WJ4)1T5
R9G)FQX
2W2)8VY
P3H)V11
243)XCC
SJT)7NF
3Y4)9SS
MP7)4ZD
186)BD5
NZ3)913
KLM)MMQ
8G9)5GZ
G38)YDV
85T)MX8
V4B)9FM
8RQ)NQ7
LX6)BMX
2SB)9MV
8KD)5VJ
J67)PGY
SD2)FC4
GWN)R2R
VL7)3W9
CB5)1TQ
5Q9)BV2
M5X)PXN
HHT)3ZB
NV4)JYQ
5TY)XQ5
RG5)NL4
CLW)Q3S
NMX)K2M
VQY)T82
FN3)CCF
TQQ)KGT
6MZ)KRF
N36)49V
Z8B)LSY
69H)M88
DX6)Y84
HQ7)1KQ
F1V)D17
JQM)8M4
QNV)Z5Z
NQ5)YJL
GSW)DDZ
6WX)3S2
CNV)T5Z
2JS)366
5JP)VPV
6LJ)6GB
YFT)347
GTS)J1D
KC9)S38
LBX)H8G
4NY)2KP
WHW)2D8
W25)9M2
R58)D8J
D17)88Z
8T7)8ZZ
7YN)HY6
ZBW)WB3
ZSC)DZK
X2B)R9B
VP7)GLT
2Q1)ZJ5
ZV3)T68
MTH)39P
SNY)4LF
2WL)JBN
WPL)D49
CCF)VVH
HPS)9M9
FYV)PQV
9N8)VMQ
92F)1DY
RWF)ZGM
CYL)V6K
3Z5)2Q1
VCN)HGD
NV8)LD6
KKV)V8H
6PZ)KVK
5VJ)KKP
GDT)NX1
CWW)4JW
Y9K)7ZT
DB4)CH5
DXB)VXC
SPD)4NV
YNV)4W3
KGT)2M2
YRJ)KV6
X9Y)TDK
S3R)L5B
8F3)7MT
XWR)TM1
Y8W)PB7
PGC)SWT
Q71)2XH
Y1X)32Q
2PD)81V
FY4)X1T
J8M)W25
WPH)2C1
N5Z)DQH
ZKY)LM8
HWD)3W8
395)429
3Q4)6NL
H4X)W8Z
WB3)MQ2
5G2)JGD
F5F)W9M
88V)HT3
GGM)J2X
FKN)P8V
VMD)3F4
RHW)9RR
RMS)243
MT8)Z8B
8YP)WW4
VLX)F82
3S2)LKC
8YS)9N8
XTX)Y2V
VGH)4W2
Z5Z)5BT
GLZ)FL6
93S)CVW
J26)ZQG
JSX)CZP
9SS)WPL
L4J)YNZ
M9J)395
5HW)8RQ
7K2)JBL
SWT)7T3
57W)Y8W
V21)WCK
9M9)NDZ
KVD)FY4
8WJ)1KH
W7K)HN3
72N)M6X
C66)1VL
JJ8)4ZS
6M9)QT9
RK6)BKR
7HP)S3V
H6F)XDR
4ZS)XZJ
R8W)4G3
6NL)H72
BGL)JP1
V5V)5G2
9M2)13Z
W7P)M28
BNW)V6V
P94)RG5
5G2)78H
2C1)HB1
ZGM)7VK
CBM)6FM
5Y3)NR2
FV1)85T
JTT)H8N
6C8)SGN
Y9M)BXH
877)DTS
JP1)TL3
D46)C5Z
2XP)XY7
H72)3VX
26Z)95S
RMV)QBH
69H)ZRT
2KV)897
4MK)JF2
P7W)DGH
RDQ)LX6
2DL)MP8
1PZ)GDT
ZTV)NVQ
KVV)NZC
22Z)9NT
B4W)S5Z
6MB)52R
B19)V8M
VRD)SDT
8ZZ)8Z4
WKG)9HB
R2R)MD3
VTP)CB5
T5Z)J3Y
KVK)QCV
DTS)23W
PCP)2X2
HN1)8KT
LGX)KKV
XG8)JFT
H1W)KZT
B1N)3Q4
NQ7)9JW
FWH)TCR
JBR)SSB
SX6)R3L
QCV)CNV
H29)QFX
TM1)2YM
ZZS)HZF
9VX)PYP
Y7D)V4B
TD8)J3R
82P)WXH
855)P2R
3W8)92F
BQZ)8WJ
VTR)JQM
NR2)5TY
L65)N34
64H)YL2
1BY)TPK
Y14)XS7
4KM)2BC
5H1)MCJ
T68)LBF
H83)SX6
HT6)C78
7W8)TFL
32T)QXX
TFL)FKP
CT7)5HW
QXP)NZ3
8C3)6J8
5GZ)FBY
V6K)9DJ
NWL)5W2
W74)ZTV
429)369
WYZ)Q7P
LJF)F5F
NVQ)S79
X7P)1TG
4CK)7X4
N9H)4NY
15X)H8Y
BV2)95F
WK6)9RM
JF2)46C
DNH)RZQ
LMN)GCR
CGB)YDQ
NXF)5H1
8RF)TVM
3VT)LFJ
LMY)2RG
7HM)C8R
GLB)MF8
5NR)QMS
CLP)V76
2W7)NYD
4W2)4PL
JBN)GPF
J3Y)XWR
C5Z)MW5
SPZ)65F
PWT)LNP
X2M)5Q9
CH5)RMV
FBY)ZZK
8Z4)R46
XH9)HT6
FDN)76V
NLM)HCM
MP8)YDX
R9B)SBW
1TQ)963
H8Y)CGB
5J4)FM6
9NB)3VT
XTZ)3Y4
CCN)714
TCR)SRX
3W9)WVL
2DL)JBR
XBV)PBL
S38)B8B
SZT)Z78
GK1)CZC
B3D)QHP
ZT8)DNB
TDK)KVV
HBT)MXC
VPV)ZTL
Q7P)NPD
QYZ)6WP
2KP)KGS
LQP)XFQ
LSG)8TQ
P62)72W
CPM)57W
2XH)XLN
R3L)DNY
TPX)X4M
KM8)64L
ZV6)82P
4NV)8N9
JP8)FYV
F8M)38T
64L)NZJ
QCV)VML
SBW)QNV
YDQ)2WD
T9F)GZ3
Z9B)8YP
KY6)2X1
TJH)LMS
YL4)W53
3CS)1JL
CVW)9LN
7DX)VTP
53M)W1H
NTB)V4W
PCP)TH8
SLL)ZZS
MJT)42C
62Y)DWD
WGC)7CB
XQ5)LMM
NKG)P97
NRK)F1V
BJL)4Z3
7YW)6MS
WHP)6F2
N7X)ZKG
WY4)JJK
NZC)G38
GBQ)BGL
8DN)3JZ
641)72N
XD4)B1N
WNT)6CF
92F)HPS
GYJ)HQP
P1W)Q17
R9D)5PC
PDZ)RHX
2CY)Z19
W1Y)5M4
32Q)2SH
BSM)NRK
QHZ)9G3
MBD)36J
TT4)NSF
PRG)VQY
4GQ)QH4
4MM)3WG
382)HC7
V48)8T7
CHG)J8M
N2M)NBS
2JD)PDS
SVG)5Y3
T9T)QFT
52R)T9F
9T5)2XV
8ZY)1SV
RL4)VHR
S6H)1TC
MGJ)TPX
WR9)SQG
6N1)XFV
DNY)X7P
TYT)HHT
XFQ)2Y4
KZT)X9Y
J1D)4KM
Y2V)W66
NC5)N9H
MGP)2GV
88Z)37G
LCW)S7P
XJL)Q4G
9G3)KL2
DZK)CNS
NJT)BS7
SC7)K19
HVS)SZT
P7L)14G
NTB)YNV
1NH)8LD
WNS)8VL
Q17)N5M
MQT)LPW
PGY)1FH
78H)M92
8ZV)BLS
FSB)BX1
9FM)9MM
FPL)GG6
29M)9NY
P76)8SN
COM)PWT
9DJ)8F3
LVQ)Y9M
C2R)SDL
6NJ)WGC
4VX)RYG
8M7)63W
MZF)59Q
61G)895
25F)W7K
28V)9WT
ZZK)T2K
L6Q)19N
NFK)P3H
B3G)CN5
P2R)1XZ
NQG)95L
HY6)1NH
BSZ)CNH
4CC)H6R
JX8)8KD
91Q)YCK
5SJ)B3D
KV6)57T
JXV)4VX
VS6)KB9
NQG)QWF
FMR)RG6
DDZ)MMW
B2Q)X4H
YMZ)PCP
MMM)BNR
SZL)36D
289)G2K
YT8)TT4
9HC)TTF
QVC)TC8
VMF)FNP
CL6)Q4N
95L)SNY
Q4G)R61
8M4)B57
JYQ)C2R
VDR)3F9
4G3)C66
R64)BQZ
X34)QF1
DQW)XJL
GPB)QM4
C3V)CPM
4J7)66R
95S)37D
B57)ZKR
78D)61G
CYN)NC5
CMP)FKN
HQ3)VCN
CZC)JSX
K8V)G8T
KT9)WBS
VZH)Y6J
9H6)PVN
CCP)3M8
VP7)DB4
KXM)TQG
5KN)Z2Y
QL2)LF3
9NY)FXS
8VY)M8K
JBS)B4W
DZD)P4N
RQF)3Z5
3Q6)WRS
GXX)5NR
63W)3QB
W8Z)J3X
6VH)VJ6
4BW)YNF
MC3)TL6
SLD)J48
MYP)HQ7
DT6)G8J
GPF)1PY
6PP)KFB
GZ3)76P
CHJ)3GM
DX1)NVB
7LG)8ZY
QF1)HQ3
85T)7DX
6T3)K7Q
49V)4BW
Y3R)DJF
4HL)641
FYC)PGC"""
def do():
splitInput = input.split()
orbits = getOrbits(splitInput)
count = countOrbits(orbits)
return [orbits, count]
def getOrbits(splitInput):
orbits = {}
for orbitDescription in splitInput:
center, orbit = orbitDescription.split(')')
orbits[orbit] = center
return orbits
def countOrbits(orbits):
count = 0
for key in orbits.keys():
currentOrbit = key
while currentOrbit:
currentOrbit = orbits.get(currentOrbit, 0)
if currentOrbit:
count += 1
return count
def countSingleOrbit(orbits, orbit):
count = 0
currentOrbit = orbit
while currentOrbit:
currentOrbit = orbits.get(currentOrbit, None)
if currentOrbit:
count += 1
return count
def move(orbit1, orbit2, commonOrbit):
countSan = countSingleOrbit(orbits, orbit1)
countYou = countSingleOrbit(orbits, orbit2)
countDouble = countSingleOrbit(orbits, commonOrbit)
return countSan + countYou - 2*countDouble - 2
def orbitList(orbits, orbit):
orbitList = []
currentOrbit = orbit
while currentOrbit:
currentOrbit = orbits.get(currentOrbit, None)
if currentOrbit:
orbitList.append(currentOrbit)
return orbitList
def findCommonOrbit(orbitList1, orbitList2):
for orbit in orbitList1:
if orbit in orbitList2:
return orbit
def do2(orbits):
orbitList1 = orbitList(orbits, 'SAN')
orbitList2 = orbitList(orbits, 'YOU')
commonOrbit = findCommonOrbit(orbitList1, orbitList2)
return move('SAN', 'YOU', commonOrbit)
orbits, count = do()
#part1
print(count)
#part2
print(do2(orbits))
<file_sep>/2019/day2.py
input = "1,0,0,3,1,1,2,3,1,3,4,3,1,5,0,3,2,1,9,19,1,19,5,23,1,13,23,27,1,27,6,31,2,31,6,35,2,6,35,39,1,39,5,43,1,13,43,47,1,6,47,51,2,13,51,55,1,10,55,59,1,59,5,63,1,10,63,67,1,67,5,71,1,71,10,75,1,9,75,79,2,13,79,83,1,9,83,87,2,87,13,91,1,10,91,95,1,95,9,99,1,13,99,103,2,103,13,107,1,107,10,111,2,10,111,115,1,115,9,119,2,119,6,123,1,5,123,127,1,5,127,131,1,10,131,135,1,135,6,139,1,10,139,143,1,143,6,147,2,147,13,151,1,5,151,155,1,155,5,159,1,159,2,163,1,163,9,0,99,2,14,0,0"
#input = "1,0,0,0,99"
def inputSplit(input):
return input.split(',')
def do(noun, verb):
splitInput = inputSplit(input)
intInput = convertToInt(splitInput)
intInput[1] = noun
intInput[2] = verb
positions = range(0, len(input) - 1, 4)
for position in positions:
action = intInput[position]
if action == 99:
return intInput
arg1 = intInput[intInput[position + 1]]
arg2 = intInput[intInput[position + 2]]
resultPos = intInput[position + 3]
if action == 1:
result = arg1 + arg2
elif action == 2:
result = arg1 * arg2
else:
print("Failure")
return
intInput[resultPos] = result
def convertToInt(input):
numbers = []
for stringNumber in input:
numbers.append(int(stringNumber))
return numbers
for noun in range(0,99):
for verb in range(0,99):
if do(noun, verb)[0] == 19690720:
print(100 * noun + verb)<file_sep>/2018/day11.py
def calculateGreatestPower(dic, kernel):
indexX = -1
indexY = -1
powerSums = [[0 for x in range(len(dic) - kernel)] for y in range(len(dic[0]) - kernel)]
maxPowerSum = -10000
for y in range(len(dic) - kernel):
for x in range(len(dic[0]) - kernel):
powerSum = 0
for i in range(kernel):
for j in range(kernel):
powerSum += dic[y + i][x + j]
powerSums[y][x] = powerSum
if powerSum > maxPowerSum:
maxPowerSum = powerSum
indexX = x
indexY = y
return [indexX + 1,indexY + 1, maxPowerSum]
def do():
serial = 5034
width = 300
height = 300
kernelwidth = 3
powermatrix = [[0 for x in range(width)] for y in range(height)]
for y in range(1, height + 1):
for x in range(1, width + 1):
rackID = x + 10
powerlevel = (rackID * y + serial) * rackID
powermatrix[y-1][x-1] = int((powerlevel % 1000) / 100) - 5
maxPower = -10000
size = 0
topleft = []
for i in range(1,301):
topleftX, topleftY, powerSum = calculateGreatestPower(powermatrix, i)
if powerSum > maxPower:
maxPower = powerSum
size = i
topleft = [topleftX, topleftY]
return [topleft, size]
print(do())<file_sep>/2018/day9_test.py
import unittest
from day9 import do
class Day9Test(unittest.TestCase):
def test1(self):
self.assertEqual(do(10, 1618), 8317)
def test2(self):
self.assertEqual(do(13, 7999), 146373)
def test3(self):
self.assertEqual(do(17, 1104), 2764)
def test4(self):
self.assertEqual(do(21, 6111), 54718)
def test5(self):
self.assertEqual(do(30, 5807), 37305)
if __name__ == '__main__':
unittest.main()
<file_sep>/2021/day4.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
def do1(splitInput):
numbers = convertToInt(splitInput[0].split(','))
boards = splitInput[1:]
intBoards = prepareBoards(boards)
return play(numbers, intBoards, True)
def do2(splitInput):
numbers = convertToInt(splitInput[0].split(','))
boards = splitInput[1:]
intBoards = prepareBoards(boards)
return play(numbers, intBoards, False)
def prepareBoards(boards):
intBoards = []
for board in boards:
splitBoard = board.split('\n')
intSplitBoard = []
for line in splitBoard:
intSplitBoard.append(convertToInt(line.split(' ')))
intBoards.append(intSplitBoard)
return intBoards
def convertToInt(input):
numbers = []
for stringNumber in input:
if stringNumber == '' :
continue
else:
numbers.append(int(stringNumber))
return numbers
def play(numbers, boards, firstWins):
for number in numbers:
for board in boards:
playNumberOnBoard(board, number)
winners = winningBoards(boards)
if winners:
if firstWins or len(boards) == 1:
return number * winningScore(boards[winners[0]])
for winner in sorted(winners, reverse=True):
boards.pop(winner)
def playNumberOnBoard(board, number):
for line in board:
if number in line:
index = line.index(number)
line[index] = 'X'
def winningBoards(boards):
winning = []
for number,board in enumerate(boards):
if ['X', 'X', 'X', 'X', 'X'] in board:
winning.append(number)
else:
for i in range(len(board[0])):
completeLine = True
for j in range(len(board)):
if board[j][i] != 'X':
completeLine = False
break
if completeLine:
winning.append(number)
break
return winning
def winningScore(board):
winningSum = 0
for line in board:
winningSum += sum([x for x in line if x != 'X'])
return winningSum
def do():
strInput = readInputFile(4)
splitInput = strInput.split('\n\n')
print(do1(splitInput))
print(do2(splitInput))
print('done')
do()<file_sep>/2021/day12.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
from collections import defaultdict
def do1(splitInput):
paths = preparePaths(splitInput)
ways = findPossibleWays(paths, 'start', 'end', [], maxAmount=defaultdict(lambda: 1))
return len(ways)
def do2(splitInput):
paths = preparePaths(splitInput)
maxAmount = {}
lowerKeys = [x for x in paths.keys() if x.islower()]
for key in lowerKeys:
maxAmount[key] = 1
allWays = []
for key in lowerKeys:
if key == 'start' or key == 'end':
continue
maxAmount[key] = 2
ways = findPossibleWays(paths, 'start', 'end', [], maxAmount)
for way in ways:
if way not in allWays:
allWays.append(way)
maxAmount[key] = 1
return len(allWays)
def preparePaths(splitInput):
paths = defaultdict(lambda: set())
for line in splitInput:
first,second = line.split('-')
firstSet = {first}
secondSet = {second}
paths[first] = paths[first].union(secondSet)
paths[second]= paths[second].union(firstSet)
return paths
def findPossibleWays(paths, start, end, path, maxAmount):
path = path + [start]
if start == end:
return [path]
newPaths = []
for connected in paths[start]:
if connected.isupper() or path.count(connected) < maxAmount[connected]:
possiblePaths = findPossibleWays(paths, connected, end, path, maxAmount)
for possiblePath in possiblePaths:
newPaths.append(possiblePath)
return newPaths
def do():
strInput = readInputFile(12)
splitInput = strInput.split('\n')
print(do1(splitInput))
print(do2(splitInput))
print('done')
do()<file_sep>/2020/day22.py
from HelperFunctions import inputsplit
from HelperFunctions import convertToInt
def do1(puzzleInput):
player1 = convertToInt(puzzleInput[0].split('\n')[1:])
player2 = convertToInt(puzzleInput[1].split('\n')[1:])
winner = play(player1, player2)
winningScore = getWinningScore(winner)
return winningScore
def do2(puzzleInput):
player1 = convertToInt(puzzleInput[0].split('\n')[1:])
player2 = convertToInt(puzzleInput[1].split('\n')[1:])
memory = []
winner = playRecursive(player1, player2, memory)
if winner == 1:
return getWinningScore(player1)
else:
return getWinningScore(player2)
def play(player1, player2):
while True:
card1 = player1.pop(0)
card2 = player2.pop(0)
if card1 < card2:
player2.append(card2)
player2.append(card1)
else:
player1.append(card1)
player1.append(card2)
if len(player1) == 0:
return player2
if len(player2) == 0:
return player1
def playRecursive(player1, player2, memory):
while True:
if (player1, player2) in memory:
return 1
else:
memory.append((player1.copy(), player2.copy()))
card1 = player1.pop(0)
card2 = player2.pop(0)
if len(player1) >= card1 and len(player2) >= card2:
winner = playRecursive(player1[0:card1].copy(), player2[0:card2].copy(), [])
else:
if card1 < card2:
winner = 2
else:
winner = 1
if winner == 1:
player1.append(card1)
player1.append(card2)
else:
player2.append(card2)
player2.append(card1)
if len(player1) == 0:
return 2
if len(player2) == 0:
return 1
def getWinningScore(player):
value = range(len(player), 0, -1)
score = sum([a*b for a,b in zip(player, value)])
return score
def do():
with open ('Input/day22.txt') as f:
strInput = f.read()
splitInput = inputsplit(strInput, '\n\n')
print(do1(splitInput))
print(do2(splitInput))
do()<file_sep>/2021/day16.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
hex = {'0': '0000', '1': '0001', '2': '0010', '3': '0011', '4': '0100', '5': '0101', '6': '0110', '7': '0111', '8': '1000', '9': '1001', 'A': '1010', 'B': '1011', 'C': '1100', 'D': '1101', 'E': '1110', 'F': '1111'}
class Package:
def __init__(self, version, typeID):
self.size = 0
self.number = 0
self.packages = []
self.version = version
self.typeID = typeID
def setSize(self, size):
self.size = size
def getSize(self):
return self.size
def setNumber(self, number):
self.number = number
def getNumber(self):
return self.number
def addPackage(self, package):
self.packages.append(package)
def setPackages(self, packages):
self.packages = packages
def getPackages(self):
return self.packages
def getVersion(self):
return self.version
def getTypeID(self):
return self.typeID
def do1(splitInput):
binaryStr = ''
for bit in splitInput:
binaryStr += hex[bit]
package = decodePackage(binaryStr)
versions = getAllVersionNumbers(package, [])
return sum(versions)
def do2(splitInput):
binaryStr = ''
for bit in splitInput:
binaryStr += hex[bit]
package = decodePackage(binaryStr)
result = calculateResult(package)
return result
def getAllVersionNumbers(package, versions):
versions.append(package.getVersion())
for subPackage in package.getPackages():
getAllVersionNumbers(subPackage, versions)
return versions
def calculateResult(package):
match package.getTypeID():
case 0:
result = 0
for subPackage in package.getPackages():
result += calculateResult(subPackage)
return result
case 1:
result = 1
for subPackage in package.getPackages():
result *= calculateResult(subPackage)
return result
case 2:
values = []
for subPackage in package.getPackages():
values.append(calculateResult(subPackage))
return min(values)
case 3:
values = []
for subPackage in package.getPackages():
values.append(calculateResult(subPackage))
return max(values)
case 4:
return package.getNumber()
case 5:
subPackages = package.getPackages()
firstValue = calculateResult(subPackages[0])
secondValue = calculateResult(subPackages[1])
if firstValue > secondValue:
return 1
else:
return 0
case 6:
subPackages = package.getPackages()
firstValue = calculateResult(subPackages[0])
secondValue = calculateResult(subPackages[1])
if firstValue < secondValue:
return 1
else:
return 0
case 7:
subPackages = package.getPackages()
firstValue = calculateResult(subPackages[0])
secondValue = calculateResult(subPackages[1])
if firstValue == secondValue:
return 1
else:
return 0
case _:
print('TypeID unknown')
return -1
def decodePackage(binaryStr):
version = int(binaryStr[0:3], 2)
typeID = int(binaryStr[3:6], 2)
package = Package(version, typeID)
match typeID:
case 4:
size,number = decodeLiteral(binaryStr[6:])
package.setSize(size)
package.setNumber(number)
case _:
lengthType, subPackages = getSubPackages(binaryStr[6:])
package.setPackages(subPackages)
size = 7
if lengthType == 1:
size += 11
else:
size += 15
for subPackage in subPackages:
size += subPackage.getSize()
package.setSize(size)
return package
def decodeLiteral(binaryStr):
notEnded = True
binaryNumber = ''
position = 0
numberOfParts = 0
while notEnded:
numberOfParts += 1
firstBit = binaryStr[position]
position += 1
if firstBit == '0':
notEnded = False
binaryNumber += binaryStr[position: (position + 4)]
position += 4
totalSize = 6 + numberOfParts * 5
return totalSize,int(binaryNumber, 2)
def getSubPackages(binaryStr):
lengthType = int(binaryStr[0],2)
packages = []
match lengthType:
case 1:
# number of subpackets
numberOfPackages = int(binaryStr[1:12], 2)
position = 12
for i in range(numberOfPackages):
package = decodePackage(binaryStr[position:])
packages.append(package)
position += package.getSize()
case 0:
# length of subpackages
lengthOfSubpackages = int(binaryStr[1:16], 2)
position = 16
while position < 16 + lengthOfSubpackages:
package = decodePackage(binaryStr[position:])
packages.append(package)
position += package.getSize()
return lengthType, packages
def do():
strInput = readInputFile(16)
print(do1(strInput))
print(do2(strInput))
print('done')
do()<file_sep>/2021/day1.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
def do1(splitInput):
bigger = [(x,y) for (x,y) in zip(splitInput, splitInput[1:]) if y > x]
return len(bigger)
def do2(splitInput):
window = [a + b + c for (a,b,c) in zip(splitInput, splitInput[1:], splitInput[2:])]
return do1(window)
def do():
strInput = readInputFile(1)
splitInput = strInput.split('\n')
intInput = convertToInt(splitInput)
print(do1(intInput))
print(do2(intInput))
do()
<file_sep>/2022/day7.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
import re
tree = {}
dirSizes = {}
fileSizes ={}
parentDir = {}
def do1(splitInput):
buildFileSystemTree(splitInput)
calculateSizes('/')
smallDirs = sum([value for value in dirSizes.values() if value <= 100000])
return smallDirs
def do2(splitInput):
unUsedSpace = 70000000 - dirSizes['/']
neededSpace = 30000000 - unUsedSpace
possibleDirs = [value for value in dirSizes.values() if value >= neededSpace]
return min(possibleDirs)
def buildFileSystemTree(splitInput):
currentDirectory = '/'
for line in splitInput:
match line.split(' '):
case ['$', 'cd', '..']:
currentDirectory = parentDir[currentDirectory]
case ['$','cd', '/']:
currentDirectory = '/'
case ['$','cd', directory]:
currentDirectory = currentDirectory + '/' + directory
case ['$', 'ls']:
tree[currentDirectory] = []
case ['dir', folder]:
tree[currentDirectory].append(currentDirectory + '/' + folder)
parentDir[currentDirectory + '/' + folder] = currentDirectory
case [size, name]:
tree[currentDirectory].append(currentDirectory + '/' + name)
fileSizes[currentDirectory + '/' + name] = int(size)
def calculateSizes(key):
if key in fileSizes.keys():
return fileSizes[key]
else:
dirSizes[key] = 0
for child in tree[key]:
dirSizes[key] += calculateSizes(child)
return dirSizes[key]
def do():
strInput = readInputFile(7)
splitInput = strInput.split('\n')
print(do1(splitInput))
print(do2(splitInput))
print('done')
do()<file_sep>/2020/day7.py
from HelperFunctions import inputsplit
import re
bagCounts = {}
def do1(puzzleInput):
bags = getBags(puzzleInput, False)
bagsToHoldAShinyGoldBag = []
for bag in bags.keys():
if containsShinyBag(bags, bag):
bagsToHoldAShinyGoldBag.append(bag)
return len(bagsToHoldAShinyGoldBag)
def do2(puzzleInput):
bags = getBags(puzzleInput, True)
return bagCount('shiny gold', bags)
def getBags(puzzleInput, numberIsImportant):
bags = {}
for line in puzzleInput:
match1 = re.search('(.+) bags contain', line)
match2 = re.findall('([0-9]+) (\D+) bag[s]?[\.|, ]', line)
bag = match1.groups()[0]
bags[bag] = []
for innerBag in match2:
if numberIsImportant:
for _ in range(int(innerBag[0])):
bags[bag].append(innerBag[1])
else:
bags[bag].append(innerBag[1])
return bags
def bagCount(bag, bags):
if bag in bagCounts.keys():
return bagCounts[bag]
count = len(bags[bag])
for innerbag in bags[bag]:
count += bagCount(innerbag, bags)
bagCounts[bag] = count
return count
def containsShinyBag(bags, bag):
if 'shiny gold' in bags[bag]:
return True
for innerBag in bags[bag]:
if containsShinyBag(bags, innerBag):
return True
return False
def do():
with open ('Input/day7.txt') as f:
strInput = f.read()
splitInput = inputsplit(strInput, '\n')
print(do1(splitInput))
print(do2(splitInput))
do()<file_sep>/2022/day22.py
from enum import IntEnum
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
class Direction(IntEnum):
NORTH = 3
EAST = 0
SOUTH = 1
WEST = 2
turnRight = {Direction.NORTH:Direction.EAST, Direction.EAST:Direction.SOUTH, Direction.SOUTH:Direction.WEST, Direction.WEST:Direction.NORTH}
turnLeft = {Direction.NORTH:Direction.WEST, Direction.EAST:Direction.NORTH, Direction.SOUTH:Direction.EAST, Direction.WEST:Direction.SOUTH}
east = {1: lambda x,y: (2,x,0,Direction.EAST), 2: lambda x,y: (5,49-x,49,Direction.WEST), 3: lambda x,y: (2,49,x,Direction.NORTH),4: lambda x,y: (5,x,0,Direction.EAST), 5: lambda x,y: (2,49-x,49,Direction.WEST), 6: lambda x,y: (5,49,x,Direction.NORTH)}
south = {1: lambda x,y: (3,0,y,Direction.SOUTH), 2: lambda x,y: (3,y,49,Direction.WEST), 3: lambda x,y: (5,0,y,Direction.SOUTH),4: lambda x,y: (6,0,y,Direction.SOUTH), 5: lambda x,y: (6,y,49,Direction.WEST), 6: lambda x,y: (2,0,y,Direction.SOUTH)}
north = {1:lambda x,y: (6,y,0,Direction.EAST), 2: lambda x,y: (6,49,y,Direction.NORTH), 3: lambda x,y: (1,49,y,Direction.NORTH), 4: lambda x,y: (3,y,0,Direction.EAST), 5: lambda x,y: (3,49,y,Direction.NORTH),6: lambda x,y: (4,49,y,Direction.NORTH)}
west = {1:lambda x,y: (4,49-x,0,Direction.EAST), 2: lambda x,y: (1,x,49,Direction.WEST), 3: lambda x,y: (4,0,x,Direction.SOUTH), 4: lambda x,y: (1,49-x,0,Direction.EAST), 5: lambda x,y: (4,x,49,Direction.WEST), 6: lambda x,y: (1,0,x,Direction.SOUTH)}
def do1(splitInput):
mapInput,steps = splitInput
map = createMap(mapInput)
startY = min([y for (x,y) in map.keys() if x == 0])
position = (0,startY)
direction = Direction.EAST
length = ''
for char in steps:
if char == 'R' or char == 'L':
position = move(int(length),position,direction,map)
length = ''
direction = turn(char, direction)
else:
length += char
position = move(int(length),position,direction,map)
return 1000 * (position[0] + 1) + 4 * (position[1] + 1) + int(direction)
def do2(splitInput):
mapInput,steps = splitInput
map,cube = createCube(mapInput)
position = (1,0,0)
direction = Direction.EAST
length = ''
for char in steps:
if char == 'R' or char == 'L':
side,posX,posY,direction = moveCube(int(length),position,direction,map,cube)
position = (side,posX,posY)
length = ''
direction = turn(char, direction)
else:
length += char
side,posX,posY,direction = moveCube(int(length),position,direction,map,cube)
mapPosX,mapPosY = cube[side][(posX,posY)]
return 1000 * (mapPosX + 1) + 4 * (mapPosY + 1) + int(direction)
def createMap(mapInput):
map = {}
for lineNumber,line in enumerate(mapInput.split('\n')):
for position,tile in enumerate(line):
if not tile == ' ':
map[(lineNumber,position)] = tile
return map
def createCube(mapInput):
map = createMap(mapInput)
cube = {1:{}, 2:{}, 3:{}, 4:{}, 5:{}, 6:{}}
x = 0
for j in range(0,50):
y = 0
for i in range(50, 100):
cube[1][x,y] = (j,i)
y += 1
y = 0
for i in range(100, 150):
cube[2][x,y] = (j,i)
y += 1
x += 1
x = 0
for j in range(50,100):
y = 0
for i in range(50, 100):
cube[3][x,y] = (j,i)
y += 1
x += 1
x = 0
for j in range(100,150):
y = 0
for i in range(0,50):
cube[4][x,y] = (j,i)
y += 1
y = 0
for i in range(50,100):
cube[5][x,y] = (j,i)
y += 1
x += 1
x = 0
for j in range(150,200):
y = 0
for i in range(0,50):
cube[6][x,y] = (j,i)
y += 1
x += 1
return map,cube
def turn(direction, currentDirection):
if direction == 'R':
return turnRight[currentDirection]
else:
return turnLeft[currentDirection]
def move(steps,position,direction,map):
currentPos = position
match direction:
case Direction.NORTH:
for _ in range(steps):
posX,posY = currentPos
nextPos = (posX - 1, posY)
if nextPos not in map.keys():
nextPosX = max([x for (x,y) in map.keys() if y == posY])
nextPos = (nextPosX,posY)
if map[nextPos] == '#':
return currentPos
else:
currentPos = nextPos
return currentPos
case Direction.EAST:
for _ in range(steps):
posX,posY = currentPos
nextPos = (posX, posY + 1)
if nextPos not in map.keys():
nextPosY = min([y for (x,y) in map.keys() if x == posX])
nextPos = (posX, nextPosY)
if map[nextPos] == '#':
return currentPos
else:
currentPos = nextPos
return currentPos
case Direction.SOUTH:
for _ in range(steps):
posX,posY = currentPos
nextPos = (posX + 1, posY)
if nextPos not in map.keys():
nextPosX = min([x for (x,y) in map.keys() if y == posY])
nextPos = (nextPosX,posY)
if map[nextPos] == '#':
return currentPos
else:
currentPos = nextPos
return currentPos
case Direction.WEST:
for _ in range(steps):
posX,posY = currentPos
nextPos = (posX, posY - 1)
if nextPos not in map.keys():
nextPosY = max([y for (x,y) in map.keys() if x == posX])
nextPos = (posX, nextPosY)
if map[nextPos] == '#':
return currentPos
else:
currentPos = nextPos
return currentPos
def moveCube(steps,position,direction,map,cube):
currentPos = position
for _ in range(steps):
match direction:
case Direction.NORTH:
side,posX,posY = currentPos
newSide = side
nextPosX,nextPosY = (posX - 1, posY)
if nextPosX < 0:
newSide,nextPosX,nextPosY,direction = north[side](posX,posY)
if map[cube[newSide][(nextPosX,nextPosY)]] == '#':
return side,posX,posY,Direction.NORTH
else:
currentPos = (newSide,nextPosX,nextPosY)
case Direction.EAST:
side,posX,posY = currentPos
newSide = side
nextPosX,nextPosY = (posX, posY + 1)
if nextPosY > 49:
newSide,nextPosX,nextPosY,direction = east[side](posX,posY)
if map[cube[newSide][(nextPosX,nextPosY)]] == '#':
return side,posX,posY,Direction.EAST
else:
currentPos = (newSide,nextPosX,nextPosY)
case Direction.SOUTH:
side,posX,posY = currentPos
newSide = side
nextPosX,nextPosY = (posX + 1, posY)
if nextPosX > 49:
newSide,nextPosX,nextPosY,direction = south[side](posX,posY)
if map[cube[newSide][(nextPosX,nextPosY)]] == '#':
return side,posX,posY,Direction.SOUTH
else:
currentPos = (newSide,nextPosX,nextPosY)
case Direction.WEST:
side,posX,posY = currentPos
newSide = side
nextPosX,nextPosY = (posX, posY - 1)
if nextPosY < 0:
newSide,nextPosX,nextPosY,direction = west[side](posX,posY)
if map[cube[newSide][(nextPosX,nextPosY)]] == '#':
return side,posX,posY,Direction.WEST
else:
currentPos = (newSide,nextPosX,nextPosY)
side,x,y = currentPos
return side,x,y,direction
def do():
strInput = readInputFile(22)
splitInput = strInput.split('\n\n')
print(do1(splitInput))
print(do2(splitInput))
print('done')
do()<file_sep>/2021/day18.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
import re
from copy import deepcopy
def do1(splitInput):
addition = splitInput[0]
for line in splitInput[1:]:
addition = '[' + addition + ',' + line + ']'
addition = reduce(addition)
magnitude = calculateMagnitude(addition)
return magnitude
def do2(splitInput):
magnitudes = []
for i in splitInput:
for j in splitInput:
if i == j:
continue
addition = '[' + i + ',' + j + ']'
addition = reduce(addition)
magnitude = calculateMagnitude(addition)
magnitudes.append(magnitude)
return max(magnitudes)
def reduce(addition):
nestingLevel = 0
lastPosition = -1
newInput = deepcopy(addition)
repeat = True
while repeat:
nestingLevel = 0
lastPosition = -1
addition = deepcopy(newInput)
repeat = False
for position,next in enumerate(addition):
match next:
case '[':
nestingLevel += 1
if nestingLevel > 4:
left,right = findInner(addition[position:])
length = len(str(left) + str(right) + ',') + 2
nextNumberPos = findNextNumber(addition[position+length:])
newInput = addition[0:position] + '0' + addition[position + length:]
newInput = addToNextNumber(nextNumberPos, right, position, newInput)
newInput = addToLastNumber(lastPosition, left, position, newInput)
repeat = True
break
case ']':
nestingLevel -= 1
case a if a.isnumeric():
if not addition[position-1].isnumeric():
lastPosition = position
# again for big numbers
if not repeat:
for position,next in enumerate(addition):
match next:
case a if a.isnumeric():
if addition[position+1].isnumeric():
number = int(addition[position:position + 2])
splitLeft = number // 2
splitRight = (number + 1) // 2
newInput = addition[0:position] + '[' + str(splitLeft) + ',' + str(splitRight) + ']' + addition[position + 2:]
repeat = True
break
return newInput
def addToNextNumber(nextNumberPos, right, position, newInput):
if nextNumberPos == -1:
return newInput
number = ''
nextNumberStart = position + nextNumberPos + 1
currentPos = nextNumberStart
while newInput[currentPos].isnumeric():
number += newInput[currentPos]
currentPos += 1
length = len(number)
number = int(number)
newInput = newInput[0: nextNumberStart] + str(number + right) + newInput[nextNumberStart + length:]
return newInput
def addToLastNumber(lastNumberPos, left, position, newInput):
if lastNumberPos == -1:
return newInput
number = ''
currentPos = lastNumberPos
while newInput[currentPos].isnumeric():
number += newInput[currentPos]
currentPos += 1
length = len(number)
number = int(number)
newInput = newInput[0:lastNumberPos] + str(number + left) + newInput[lastNumberPos + length:]
return newInput
def findInner(addition):
left = ''
right = ''
isLeft = True
lastOpened = 0
for next in addition[1:]:
match next:
case '[':
if isLeft:
left += next
else:
right += next
lastOpened += 1
case ']':
if lastOpened == 0:
if left.isnumeric():
left = int(left)
if right.isnumeric():
right = int(right)
return left,right
if isLeft:
left += next
else:
right += next
lastOpened -= 1
case ',':
if lastOpened == 0:
isLeft = False
continue
else:
if isLeft:
left += next
else:
right += next
case _:
if isLeft:
left += next
else:
right += next
def findNextNumber(input):
for position,value in enumerate(input):
if value.isnumeric():
return position
return -1
def calculateMagnitude(addition):
if str(addition).isnumeric():
return addition
left,right = findInner(addition)
return 3* calculateMagnitude(left) + 2* calculateMagnitude(right)
def do():
strInput = readInputFile(18)
splitInput = strInput.split('\n')
print(do1(splitInput))
print(do2(splitInput))
print('done')
do()<file_sep>/2020/day17-2.py
from HelperFunctions import inputsplit
from copy import deepcopy
def do1(puzzleInput):
points = prepareInput(puzzleInput, 3)
points = changeState(points, 3)
count = countActiveCubes(points)
return count
def do2(puzzleInput):
points = prepareInput(puzzleInput, 4)
points = changeState(points, 4)
count = countActiveCubes(points)
return count
def changeState(points, dimension):
newPoints = deepcopy(points)
for _ in range(6):
points = deepcopy(newPoints)
neighbors = set()
for point in points:
directNeighbors = getNeighbors(point, points, dimension)
neighbors = neighbors.union(directNeighbors)
activeNeighbors = countActiveNeighbors(directNeighbors, points)
if not (2 <= activeNeighbors <= 3):
newPoints.remove(point)
for neighbor in neighbors:
if neighbor in points:
continue
activeNeighbors = countActiveNeighbors(getNeighbors(neighbor, points, dimension), points)
if activeNeighbors == 3:
newPoints.add(neighbor)
return newPoints
def getNeighbors(point, points, dimension):
neighbors = set()
if dimension == 3:
x,y,z = point
elif dimension == 4:
x,y,z,w = point
step = [-1, 0, 1]
for xStep in step:
for yStep in step:
for zStep in step:
if dimension == 4:
for wStep in step:
if (xStep == 0 and yStep == 0 and zStep == 0 and wStep == 0):
continue
neighbors.add((x + xStep, y + yStep, z + zStep, w + wStep))
elif dimension == 3:
if (xStep == 0 and yStep == 0 and zStep == 0):
continue
neighbors.add((x + xStep, y + yStep, z + zStep))
return neighbors
def countActiveNeighbors(neighbors, points):
activeNeighbors = [x for x in neighbors if x in points]
return len(activeNeighbors)
def prepareInput(puzzleInput, dimension):
points = set()
for x,line in enumerate(puzzleInput):
for y,cube in enumerate(line):
if cube == '#':
if dimension == 4:
points.add((x,y,0,0))
elif dimension == 3:
points.add((x,y,0))
return points
def countActiveCubes(space):
return len(space)
def do():
with open ('Input/day17.txt') as f:
strInput = f.read()
splitInput = inputsplit(strInput, '\n')
print(do1(splitInput))
print(do2(splitInput))
do()<file_sep>/2022/day6.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
def do1(strInput):
windowSize = 4
result = [y + windowSize for y,x in enumerate([strInput[i:i+windowSize] for i in range(len(strInput) - windowSize + 1)]) if len(set(x)) == windowSize][0]
return result
def do2(strInput):
windowSize = 14
result = [y + windowSize for y,x in enumerate([strInput[i:i+windowSize] for i in range(len(strInput) - windowSize + 1)]) if len(set(x)) == windowSize][0]
return result
def do():
strInput = readInputFile(6)
print(do1(strInput))
print(do2(strInput))
print('done')
do()<file_sep>/2020/day20.py
from HelperFunctions import inputsplit
import re
class Tile:
def __init__(self, number=0, upper=[], lower=[], right=[], left=[], inner=[]):
self.upper = upper.copy()
self.lower = lower.copy()
self.right = right.copy()
self.left = left.copy()
self.inner = inner
self.number = number
self.neighbors = set()
self.leftNeighbor = 0
self.rightNeighbor = 0
self.upperNeighbor = 0
self.lowerNeighbor = 0
self.fixed = False
def flip(self):
if self.fixed:
return
tmp = self.upper.copy()
self.upper = self.lower.copy()
self.lower = tmp.copy()
self.right.reverse()
self.left.reverse()
self.inner.reverse()
def rotate(self, angle):
if self.fixed:
return
if angle == 0:
return
if angle == 90:
tmp = self.upper
self.upper = self.left.copy()
self.upper.reverse()
self.left = self.lower.copy()
self.lower = self.right.copy()
self.lower.reverse()
self.right = tmp.copy()
self.rotateInner()
if angle == 180:
self.rotate(90)
self.rotate(90)
if angle == 270:
self.rotate(90)
self.rotate(90)
self.rotate(90)
def rotateInner(self):
newInner = []
for x in range(len(self.inner)):
column = []
for y in range(len(self.inner)):
column.append(self.inner[y][x])
column.reverse()
newInner.append(column)
self.inner = newInner.copy()
def checkForEqualSides(self, otherTile):
if self.upper == otherTile.lower:
if self.fixed:
otherTile.fixed = True
self.upperNeighbor = otherTile
otherTile.lowerNeighbor = self
return True
if self.lower == otherTile.upper:
if self.fixed:
otherTile.fixed = True
self.lowerNeighbor = otherTile
otherTile.upperNeighbor = self
return True
if self.right == otherTile.left:
if self.fixed:
otherTile.fixed = True
self.rightNeighbor = otherTile
otherTile.leftNeighbor = self
return True
if self.left == otherTile.right:
if self.fixed:
otherTile.fixed = True
self.leftNeighbor = otherTile
otherTile.rightNeighbor = self
return True
return False
def fixNeighbors(self):
for neighbor in self.neighbors:
self.couldBeNeighbors(neighbor)
def couldBeNeighbors(self, otherTile):
flipped = False
while True:
for angle in [0, 90, 180, 270]:
otherTile.rotate(angle)
if self.checkForEqualSides(otherTile):
self.neighbors.add(otherTile)
return True
otherTile.flip()
if flipped:
break
flipped = True
return False
def do1(puzzleInput):
tiles = extractTiles(puzzleInput)
neighbors = getNeighbors(tiles)
multiplied = 1
for key,neighbors in neighbors.items():
if len(neighbors) == 2:
multiplied *= key
return multiplied
def do2(puzzleInput):
tiles = extractTiles(puzzleInput)
neighbors = getNeighbors(tiles)
corners = [x for x in tiles if len(x.neighbors) == 2]
arrange(tiles, neighbors, corners[0])
corner1 = [x for x in corners if x.rightNeighbor != 0 and x.lowerNeighbor != 0][0]
image = buildImage(tiles, corner1)
notSeaMonsters = notSeaMonsterHashes(image)
return notSeaMonsters
def notSeaMonsterHashes(image):
imageTile = Tile(inner=image)
numberOfHashes = 0
for line in imageTile.inner:
numberOfHashes += line.count('#')
middleLine = '#[\.\#]{4}##[\.\#]{4}##[\.\#]{4}###'
upperLine = '[\.\#]{18}#[\.\#]{1}'
lowerLine = '[\.\#]{1}#[\.\#]{2}#[\.\#]{2}#[\.\#]{2}#[\.\#]{2}#[\.\#]{2}#[\.\#]{3}'
rotated = False
while True:
for rotationAngle in [0, 90, 180, 270]:
seaMonsters = 0
imageTile.rotate(rotationAngle)
for index,line in enumerate(imageTile.inner):
s = ''
imageTile.inner[index] = s.join(line)
for index,line in enumerate(imageTile.inner[0:-1]):
matchMiddle = re.search(middleLine, line)
if matchMiddle:
startPos = matchMiddle.start()
matchLower = re.fullmatch(lowerLine, imageTile.inner[index + 1][startPos:startPos + 20])
matchUpper = re.fullmatch(upperLine, imageTile.inner[index - 1][startPos:startPos + 20])
if matchLower and matchUpper:
seaMonsters += 1
if seaMonsters:
return numberOfHashes - seaMonsters * 15
imageTile.flip()
if rotated:
break
rotated = True
return numberOfHashes - seaMonsters * 15
def arrange(tiles, neighbors, tile):
tile.fixed = True
tile.fixNeighbors()
while any([not x.fixed for x in tiles]):
for otherTile in [x for x in tiles if x.fixed]:
otherTile.fixNeighbors()
def buildImage(tiles, corner1):
image = []
currentTile = corner1
lowerNeighbor = currentTile.lowerNeighbor
while lowerNeighbor:
imageLine = currentTile.inner.copy()
rightNeighbor = currentTile.rightNeighbor
while rightNeighbor:
for x,line in enumerate(imageLine):
imageLine[x] = line + rightNeighbor.inner[x]
rightNeighbor = rightNeighbor.rightNeighbor
for line in imageLine:
image.append(line)
currentTile = lowerNeighbor
lowerNeighbor = currentTile.lowerNeighbor
imageLine = currentTile.inner.copy()
rightNeighbor = currentTile.rightNeighbor
while rightNeighbor:
for x,line in enumerate(imageLine):
imageLine[x] = line + rightNeighbor.inner[x]
rightNeighbor = rightNeighbor.rightNeighbor
for line in imageLine:
image.append(line)
return image
def extractTiles(puzzleInput):
tiles = []
for part in puzzleInput:
lines = part.split('\n')
number = int(lines[0][5:9])
upper = list(lines[1])
lower = list(lines[-1])
left = []
right = []
for line in lines[1:]:
left.append(line[0])
right.append(line[-1])
inner = []
for line in lines[2:-1]:
inner.append(list(line[1:-1]))
tiles.append(Tile(number, upper, lower, right, left, inner))
return tiles
def getNeighbors(tiles):
neighbors = {}
for tile in tiles:
neighbors[tile.number] = []
for otherTile in tiles:
if tile == otherTile:
continue
if tile.couldBeNeighbors(otherTile):
neighbors[tile.number].append(otherTile.number)
return neighbors
def do():
with open ('Input/day20.txt') as f:
strInput = f.read()
splitInput = inputsplit(strInput, '\n\n')
print(do1(splitInput))
print(do2(splitInput))
do()<file_sep>/2021/day15.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
from collections import defaultdict
from copy import deepcopy
def do1(splitInput):
costs = getSums(splitInput)
return costs[(len(splitInput[0]) - 1, len(splitInput) - 1)]
def do2(splitInput):
newMap = generateBiggerMap(splitInput)
costs = getSums(newMap)
return costs[(len(newMap[0]) - 1, len(newMap) - 1)]
def generateBiggerMap(splitInput):
newLines = []
for line in splitInput:
line = convertToInt(line)
newPart = deepcopy(line)
for i in range(4):
newPart = [x + 1 if x < 9 else 1 for x in newPart]
line += newPart
newLines.append(line)
newMap = deepcopy(newLines)
for i in range(1, 5):
for line in newLines:
newMap.append([x + i if x + i < 10 else (x + i) - 9 for x in line])
return newMap
def getSums(splitInput):
costs = defaultdict(lambda: 1000000000)
costs[(0,0)] = 0
changed = True
while(changed):
changed = False
for lineNumber,line in enumerate(splitInput):
for position,risk in enumerate(line):
if lineNumber == 0 and position == 0:
continue
left = (lineNumber, position - 1)
right = (lineNumber, position + 1)
up = (lineNumber - 1, position)
down = (lineNumber + 1, position)
minNeighborCost = 1000000000
for neighbor in [left, right, up, down]:
minNeighborCost = min(minNeighborCost, costs[neighbor])
newCosts = int(risk) + minNeighborCost
if newCosts < costs[(lineNumber, position)]:
changed = True
costs[(lineNumber, position)] = newCosts
return costs
def do():
strInput = readInputFile(15)
splitInput = strInput.split('\n')
print(do1(splitInput))
print(do2(splitInput))
print('done')
do()<file_sep>/2021/day10.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
from collections import defaultdict
correspondingCharacters = {')':'(', ']':'[', '}':'{', '>':'<'}
correspondingCharacters_reverse = {'(':')', '[':']', '{':'}', '<':'>'}
points = {')': 3, ']': 57, '}': 1197, '>': 25137, None: 0}
points2 = {')': 1, ']': 2, '}': 3, '>': 4, None: 0}
def do1(splitInput):
score = 0
for line in splitInput:
illegalCharacter,_ = findIllegalCharacter(line)
score += points[illegalCharacter]
return score
def do2(splitInput):
scores = []
for line in splitInput:
illegalCharacter,remainingCloses = findIllegalCharacter(line)
if not illegalCharacter:
lineScore = 0
while remainingCloses:
lineScore = lineScore * 5
lineScore += points2[correspondingCharacters_reverse[remainingCloses.pop()]]
scores.append(lineScore)
scores.sort()
return scores[len(scores) // 2]
def findIllegalCharacter(line):
lastOpened = []
for character in line:
match character:
case '(' | '[' | '{' | '<':
lastOpened.append(character)
case ']' | ')' | '}' | '>' :
correspondingCharacter = correspondingCharacters[character]
if lastOpened[-1] != correspondingCharacter:
return character,lastOpened
else:
lastOpened.pop()
return None,lastOpened
def do():
strInput = readInputFile(10)
splitInput = strInput.split('\n')
print(do1(splitInput))
print(do2(splitInput))
print('done')
do()<file_sep>/2020/day9.py
from HelperFunctions import inputsplit
from HelperFunctions import convertToInt
from itertools import islice
def do1(puzzleInput, preambelLength):
for position in range(preambelLength, len(puzzleInput) - preambelLength):
splitArray = puzzleInput[(position - preambelLength):position]
possibleSums = [(x,y) for x in splitArray for y in splitArray if (x + y) == puzzleInput[position]]
if len(possibleSums) == 0:
return puzzleInput[position]
return 'nothing found'
def do2(puzzleInput, invalidNumber):
sliceSize = 2
while True:
for i in range(len(puzzleInput)):
arraySlice = puzzleInput[slice(i, i + sliceSize, 1)]
if (sum(arraySlice) == invalidNumber):
return min(arraySlice) + max(arraySlice)
sliceSize += 1
return 'nothing found'
def do():
with open ('Input/day9.txt') as f:
strInput = f.read()
splitInput = inputsplit(strInput, '\n')
intInput = convertToInt(splitInput)
preambelLength = 25
result1 = do1(intInput, preambelLength)
print(result1)
print(do2(intInput, result1))
do()<file_sep>/2020/day6.py
from HelperFunctions import inputsplit
def do1(puzzleInput):
return getGroupCount(puzzleInput, union)
def do2(puzzleInput):
return getGroupCount(puzzleInput, intersection)
def getGroupCount(puzzleInput, mergeFunction):
groupCount = 0
for group in puzzleInput:
persons = group.split('\n')
answers = set(persons[0])
for otherPerson in persons[1:]:
answer = set(otherPerson)
answers = mergeFunction(answers, answer)
groupCount += len(answers)
return groupCount
def intersection(set1, set2):
return set1.intersection(set2)
def union(set1, set2):
return set1.union(set2)
def do():
with open ('Input/day6.txt') as f:
strInput = f.read()
splitInput = inputsplit(strInput, '\n\n')
print(do1(splitInput))
print(do2(splitInput))
do()<file_sep>/2019/day10.py
import math
input =""".###.#...#.#.##.#.####..
.#....#####...#.######..
#.#.###.###.#.....#.####
##.###..##..####.#.####.
###########.#######.##.#
##########.#########.##.
.#.##.########.##...###.
###.#.##.#####.#.###.###
##.#####.##..###.#.##.#.
.#.#.#####.####.#..#####
.###.#####.#..#..##.#.##
########.##.#...########
.####..##..#.###.###.#.#
....######.##.#.######.#
###.####.######.#....###
############.#.#.##.####
##...##..####.####.#..##
.###.#########.###..#.##
#.##.#.#...##...#####..#
##.#..###############.##
##.###.#####.##.######..
##.#####.#.#.##..#######
...#######.######...####
#....#.#.#.####.#.#.#.##
"""
def do():
grid = input.split()
asteroids = getAsteroids(grid)
counts = {}
detectedAsteroids = {}
for x,y in asteroids:
count, seenAsteroids = detectAsteroids(asteroids, (x,y))
counts[(x,y)] = count
detectedAsteroids[(x,y)] = seenAsteroids
return counts, detectedAsteroids
def do2(center, asteroids):
angles = {}
upperPoint = (center[0], 0)
for asteroid in asteroids:
angle = getAngle(upperPoint, center, asteroid)
angles[asteroid] = angle
return angles
def getAsteroids(grid):
asteroids = []
for y in range(len(grid)):
line = grid[y]
for x in range(len(line)):
point = line[x]
if point != '#':
continue
asteroids.append((x,y))
return asteroids
def getAngle(a, b, c):
ang = math.degrees(math.atan2(c[1]-b[1], c[0]-b[0]) - math.atan2(a[1]-b[1], a[0]-b[0]))
return ang + 360 if ang < 0 else ang
def detectAsteroids(asteroids, center):
count = 0
seenAsteroids = []
for x,y in asteroids:
if (x,y) == center:
continue
if canBeSeen(center, asteroids, (x,y)):
count += 1
seenAsteroids.append((x,y))
return count, seenAsteroids
def canBeSeen(center, asteroids, endPoint):
canBeSeen = True
for x,y in asteroids:
if (x,y) == center or (x,y) == endPoint:
continue
if isBetween(center, endPoint, (x,y)):
canBeSeen = False
break
return canBeSeen
def isBetween(center, endPoint, point):
crossProduct = (point[1] - center[1]) * (endPoint[0] - center[0]) - (point[0] - center[0]) * (endPoint[1] - center[1])
if crossProduct != 0:
return False
dotProduct = (point[0] - center[0]) * (endPoint[0] - center[0]) + (point[1] - center[1])*(endPoint[1] - center[1])
if dotProduct < 0:
return False
squaredLength = (endPoint[0] - center[0])*(endPoint[0] - center[0]) + (endPoint[1] - center[1])*(endPoint[1] - center[1])
if dotProduct > squaredLength:
return False
return True
#part1
counts, asteroids = do()
maxKey = max(counts, key=counts.get)
maxValue = counts[maxKey]
print(maxValue)
#part2
angles = do2(maxKey, asteroids[maxKey])
sortedAngles = list((sorted(angles.items(), key=lambda item: item[1])))
print (sortedAngles[199][0][0] * 100 + sortedAngles[199][0][1])
<file_sep>/2020/day2.py
from HelperFunctions import inputsplit
import re
def do1(puzzleInput):
numValidPasswords = 0
for line in puzzleInput:
match = re.match('([0-9]+)-([0-9]+) ([A-Za-z]): ([A-Za-z]+)', line)
minCount, maxCount, letter, password = [int(x) if x.isnumeric() else x for x in match.groups()]
numberOfOccurences = password.count(letter)
if minCount <= numberOfOccurences <= maxCount:
numValidPasswords += 1
return numValidPasswords
def do2(puzzleInput):
numValidPasswords = 0
for line in puzzleInput:
match = re.match('([0-9]+)-([0-9]+) ([A-Za-z]): ([A-Za-z]+)', line)
pos1, pos2, letter, password = [int(x) - 1 if x.isnumeric() else x for x in match.groups()]
if (password[pos1] == letter and not password[pos2] == letter) or (password[pos2] == letter and not password[pos1] == letter):
numValidPasswords += 1
return numValidPasswords
def do():
with open ('Input/day2.txt') as f:
strInput = f.read()
splitInput = inputsplit(strInput, '\n')
print(do1(splitInput))
print(do2(splitInput))
do()<file_sep>/2022/day1.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
def calculateElfCalories(splitInput):
elfCalories = []
for elf in splitInput:
calories = convertToInt(elf.split('\n'))
elfCalories.append(sum(calories))
return elfCalories
def do1(splitInput):
elfCalories = calculateElfCalories(splitInput)
return max(elfCalories)
def do2(splitInput):
elfCalories = calculateElfCalories(splitInput)
elfCalories.sort(reverse=True)
return sum(elfCalories[0:3])
def do():
strInput = readInputFile(1)
splitInput = strInput.split('\n\n')
print(do1(splitInput))
print(do2(splitInput))
print('done')
do()<file_sep>/2021/day8.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
from collections import defaultdict
numberMapping = {1: ['c', 'f'], 2: ['a', 'c', 'd', 'e', 'g'], 3: ['a', 'c', 'd', 'f', 'g'], 4: ['b', 'c', 'd', 'f'], 5: ['a', 'b', 'd', 'f', 'g'], 6: ['a', 'b', 'd', 'e', 'f', 'g'], 7: ['a', 'c', 'f'], 8: ['a', 'b', 'c', 'd', 'e', 'f', 'g'], 9: ['a', 'b', 'c', 'd', 'f', 'g'], 0: ['a', 'b', 'c', 'e', 'f', 'g']}
allPossibleDigitsPerNumber = {2: ['c', 'f'], 3: ['a', 'c', 'f'], 4: ['b', 'c', 'd', 'f'], 7: ['a', 'b', 'c', 'd', 'e', 'f', 'g'], 5: ['a', 'b', 'c', 'd', 'e', 'f', 'g'], 6: ['a', 'b', 'c', 'd', 'e', 'f', 'g']}
def do1(splitInput):
uniqueDigits = 0
for line in splitInput:
input,output = line.split('|')
outputDigits = output.split(' ')
for digit in outputDigits:
if len(digit) in [2, 3, 4, 7]:
uniqueDigits += 1
return uniqueDigits
def do2(splitInput):
sumOutputValues = 0
for line in splitInput:
input,output = line.split('|')
inputValues = input.split(' ')
outputValues = output.split(' ')
mapping = getMapping(inputValues)
outputValue = calculateOutputValues(outputValues, mapping)
sumOutputValues += outputValue
return sumOutputValues
def getMapping(inputValues):
mapping = {'a': set(('a', 'b', 'c', 'd', 'e', 'f', 'g')), 'b': set(('a', 'b', 'c', 'd', 'e', 'f', 'g')), 'c': set(('a', 'b', 'c', 'd', 'e', 'f', 'g')), 'd': set(('a', 'b', 'c', 'd', 'e', 'f', 'g')), 'e': set(('a', 'b', 'c', 'd', 'e', 'f', 'g')), 'f': set(('a', 'b', 'c', 'd', 'e', 'f', 'g')), 'g': set(('a', 'b', 'c', 'd', 'e', 'f', 'g'))}
inputPerLength = defaultdict(lambda: [])
for inputValue in inputValues[0:-1]:
length = len(inputValue)
inputPerLength[length].append([x for x in inputValue])
possibleValues = set(allPossibleDigitsPerNumber[length])
for digit in inputValue:
mapping[digit].intersection_update(possibleValues)
# c & f (1)
one1,one2 = [x for x in mapping.keys() if mapping[x] == {'c', 'f'}]
sumOfOne = countOccurence(one1, inputValues)
if sumOfOne == 8:
mapping[one1] = {'c'}
removeFromMapping(one1, 'c', mapping)
removeFromMapping(one2, 'f', mapping)
else:
mapping[one1] = {'f'}
removeFromMapping(one1, 'f', mapping)
removeFromMapping(one2, 'c', mapping)
# a (7)
seven = [x for x in mapping.keys() if mapping[x] == {'a'}][0]
removeFromMapping(seven, 'a', mapping)
# b & d (4)
four1,four2 = [x for x in mapping.keys() if mapping[x] == {'b', 'd'}]
sumOfFour = countOccurence(four1, inputValues)
if sumOfFour == 6:
mapping[four1] = {'b'}
removeFromMapping(four1, 'b', mapping)
removeFromMapping(four2, 'd', mapping)
else:
mapping[four1] = {'d'}
removeFromMapping(four1, 'd', mapping)
removeFromMapping(four2, 'b', mapping)
# e & g
other1,other2 = [x for x in mapping.keys() if mapping[x] == {'e', 'g'}]
sumOfOthers = countOccurence(other1, inputValues)
if sumOfOthers == 4:
mapping[other1] = {'e'}
removeFromMapping(other1, 'e', mapping)
else:
mapping[other1] = {'g'}
removeFromMapping(other1, 'g', mapping)
return mapping
def countOccurence(digit, inputValues):
sumOfDigit = 0
for input in inputValues:
if digit in input:
sumOfDigit += 1
return sumOfDigit
def removeFromMapping(rightKey, digit, mapping):
for key in mapping.keys():
if key != rightKey:
if digit in mapping[key]:
mapping[key].remove(digit)
def calculateOutputValues(outputValues, mapping):
result = ''
for outputValue in outputValues:
if outputValue == '':
continue
resultStr = []
for digit in outputValue:
resultStr.append(list(mapping[digit])[0])
sortedResult = sorted(resultStr)
resultDigit = str([x for x in numberMapping.keys() if sorted(numberMapping[x]) == sortedResult][0])
result += resultDigit
return int(result)
def do():
strInput = readInputFile(8)
splitInput = strInput.split('\n')
print(do1(splitInput))
print(do2(splitInput))
print('done')
do()<file_sep>/2018/day9_2.py
from collections import deque
def do(players, marbles):
player = 1
marble_list = deque([0])
scores = {}
for marble in range(1, marbles + 1):
if (marble % 23 == 0):
points = marble
marble_list.rotate(7)
points += marble_list.popleft()
if player not in scores:
scores[player] = 0
scores[player] += points
else:
marble_list.rotate(-2)
marble_list.insert(0, marble)
player = (player + 1) % players
return max(scores.values())
print(do(477, 7085100))<file_sep>/2021/day19.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
import re
from collections import Counter
rotations = ['x,y,z', '-x,-y,-z', 'y,-x,z', '-y,x,-z', '-x,-y,z', 'x,y,-z', '-y,x,z', 'y,-x,-z', 'z,y,-x', '-z,-y,x', 'y,-z,-x',
'-y,z,x', '-z,-y,-x', 'z,y,x', '-y,z,-x', 'y,-z,x', 'z,-x,-y', '-z,x,y', '-x,-z,-y', 'x,z,y',
'-z,x,-y', 'z,-x,y', 'x,z,-y', '-x,-z,y', 'z,-y,x', '-z,y,-x', '-y,-z,x', 'y,z,-x', '-z,y,x', 'z,-y,-x',
'y,z,x', '-y,-z,-x', 'z,x,y', '-z,-x,-y', 'x,-z,y', '-x,z,-y', '-z,-x,y', 'z,x,-y',
'-x,z,y', 'x,-z,-y', '-x,y,-z', 'x,-y,z', 'y,x,-z', '-y,-x,z', 'x,-y,-z', '-x,y,z', '-y,-x,-z', 'y,x,z']
alreadyInScanner0 = []
scannerPositions = [(0,0,0)]
def do1(splitInput):
scannerPoints = parseInput(splitInput)
while len(alreadyInScanner0) < len(scannerPoints.keys()) - 1:
scannerOrientations = findScannerOrientation(scannerPoints)
addPointsToScanner(scannerOrientations, scannerPoints)
return len(scannerPoints[0])
def do2():
return getLargestScannerDistance()
def getLargestScannerDistance():
distances = []
for x1,y1,z1 in scannerPositions:
for x2,y2,z2 in scannerPositions:
distance = abs(x1-x2) + abs(y1-y2) + abs(z1-z2)
distances.append(distance)
return max(distances)
def getRotatedPoint(point, rotation):
x,y,z = point
match = re.match('([-]?[xyz]),([-]?[xyz]),([-]?[xyz])', rotation)
newPoint = []
for part in match.groups():
negative = 1
if len(part) > 1:
negative = -1
part = part[1]
match part:
case 'x':
newPoint.append(negative * x)
case 'y':
newPoint.append(negative * y)
case 'z':
newPoint.append(negative * z)
return (newPoint[0], newPoint[1], newPoint[2])
def addPointsToScanner(scannerOrientations, scannerPoints):
for scannerNr,position,rotation in scannerOrientations:
if scannerNr in alreadyInScanner0:
continue
xPos,yPos,zPos = position
scannerPositions.append(position)
firstScanner = set(scannerPoints[0])
secondScanner = scannerPoints[scannerNr]
for point in secondScanner:
x,y,z = getRotatedPoint(point, rotation)
newPoint = (xPos - x, yPos - y, zPos - z)
firstScanner.add(newPoint)
scannerPoints[0] = list(firstScanner)
alreadyInScanner0.append(scannerNr)
def findScannerOrientation(scannerPoints):
scannerOrientation = []
scanner1 = scannerPoints[0]
for secondScanner,scanner2 in scannerPoints.items():
if secondScanner == 0 or secondScanner in alreadyInScanner0:
continue
for rotation in rotations:
newPoints = Counter()
for point1 in scanner1:
x1,y1,z1 = point1
for point2 in scanner2:
x2,y2,z2 = getRotatedPoint(point2, rotation)
newPoint = (x1 + x2, y1 + y2, z1 + z2)
newPoints[newPoint] += 1
result = [x for x in newPoints.keys() if newPoints[x] > 10]
if result:
scannerOrientation.append((secondScanner, result[0], rotation))
return scannerOrientation
def parseInput(splitInput):
scannerPoints = {}
for scanner in splitInput:
scanner = scanner.split('\n')
match = re.match('--- scanner ([0-9]+) ---', scanner[0])
scannerNumber = int(match.groups()[0])
scannerPoints[scannerNumber] = []
for line in scanner[1:]:
x,y,z = line.split(',')
x = int(x)
y = int(y)
z = int(z)
scannerPoints[scannerNumber].append((x,y,z))
return scannerPoints
def do():
strInput = readInputFile(19)
splitInput = strInput.split('\n\n')
print(do1(splitInput))
print(do2())
print('done')
do()<file_sep>/2018/day14.py
input = 894501
def doOne():
l = [3,7]
elf1 = 0
elf2 = 1
while True:
newRecipe = l[elf1] + l[elf2]
numbers = [int(x) for x in str(newRecipe)]
for number in numbers:
l.append(number)
elf1 = (elf1 + 1 + l[elf1]) % len(l)
elf2 = (elf2 + 1 + l[elf2]) % len(l)
if len(l) >= input + 10:
return(l[input:])
def doTwo():
l = [3,7]
elf1 = 0
elf2 = 1
inputstr = [int(x) for x in str(input)]
resultIndex = 2
index = 0
while True:
newRecipe = l[elf1] + l[elf2]
numbers = [int(x) for x in str(newRecipe)]
for number in numbers:
if number == inputstr[index]:
resultIndex = len(l) - index
index += 1
if index == len(inputstr):
return resultIndex
else:
index = 0
if number == inputstr[index]:
resultIndex = len(l) - index
index += 1
if index == len(inputstr):
return resultIndex
l.append(number)
elf1 = (elf1 + 1 + l[elf1]) % len(l)
elf2 = (elf2 + 1 + l[elf2]) % len(l)
print(doOne())
print(doTwo())<file_sep>/2020/day16.py
from HelperFunctions import inputsplit
from HelperFunctions import convertToInt
from copy import deepcopy
import re
def do1(puzzleInput):
valid = puzzleInput[0].split('\n')
nearbyTickets = puzzleInput[2].split('\n')[1:]
validFields = extractValidFields(valid)
invalidValues = checkNearbyTickets(nearbyTickets, validFields)
return sum(invalidValues)
def do2(puzzleInput):
valid = puzzleInput[0].split('\n')
myTicket = puzzleInput[1].split('\n')[1]
nearbyTickets = puzzleInput[2].split('\n')[1:]
validFields = extractValidFields(valid)
validTickets = getValidTickets(nearbyTickets, validFields)
assignedFields = getActualFields(validTickets, validFields)
result = multiplyDepartureValues(assignedFields, myTicket)
return result
def extractValidFields(valid):
validFields = {}
for line in valid:
match = re.match('([a-z ]+): ([0-9]+)-([0-9]+) or ([0-9]+)-([0-9]+)', line)
name, x1, x2, y1, y2 = [int(x) if x.isnumeric() else x for x in match.groups()]
validFields[name] = []
for i in range(x1, x2 + 1):
validFields[name].append(i)
for i in range(y1, y2 + 1):
validFields[name].append(i)
return validFields
def checkNearbyTickets(tickets, validFields):
invalidValues = []
for ticket in tickets:
strfields = ticket.split(',')
fields = convertToInt(strfields)
for field in fields:
valid = False
for value in validFields.values():
if field in value:
valid = True
if not valid:
invalidValues.append(field)
return invalidValues
def getValidTickets(tickets, validFields):
validIntTickets = []
for ticket in tickets:
ticketValid = True
strfields = ticket.split(',')
fields = convertToInt(strfields)
for field in fields:
valid = False
for value in validFields.values():
if field in value:
valid = True
if not valid:
ticketValid = False
break
if ticketValid:
validIntTickets.append(fields)
return validIntTickets
def getActualFields(validTickets, validFields):
possibleFields = {}
for position in range(0, len(validTickets[0])):
possibleFields[position] = []
for key,value in validFields.items():
possibleFields[position].append(key)
for ticket in validTickets:
for position,field in enumerate(ticket):
for key in possibleFields[position]:
if not (field in validFields[key]):
possibleFields[position].remove(key)
solution = {}
while (len(solution.keys()) != len(validFields.keys())):
for key,value in possibleFields.items():
if len(value) == 1:
solution[key] = value[0]
for otherValues in possibleFields.values():
if solution[key] in otherValues:
otherValues.remove(solution[key])
return solution
def multiplyDepartureValues(assignedFields, myTicket):
myTicket = [int(x) for x in myTicket.split(',')]
result = 1
for key,value in assignedFields.items():
if re.match('departure', value):
result *= myTicket[key]
return result
def do():
with open ('Input/day16.txt') as f:
strInput = f.read()
splitInput = inputsplit(strInput, '\n\n')
print(do1(splitInput))
print(do2(splitInput))
do()<file_sep>/2022/create.py
import os
import sys
from pathlib import Path
def createFiles(filepath, filepathInput, filepathExampleInput, day):
if not Path(filepath).exists():
with open(filepath, 'w') as f:
f.writelines(['from HelperFunctions import readInputFile\n', 'from HelperFunctions import readExampleInput\n', 'from HelperFunctions import convertToInt\n\n', 'def do1(splitInput):\n',
'\treturn \'done\'\n\n', 'def do2(splitInput):\n', '\treturn \'done\'\n\n', 'def do():\n', f'\tstrInput = readInputFile({day})\n', f'\tstrInput = readExampleInput({day})\n\n',
'\tprint(do1(strInput))\n', '\tprint(do2(strInput))\n\n', '\tprint(\'done\')\n\n\n', 'do()'])
if not Path(filepathInput).exists():
open(filepathInput, 'a').close()
if not Path(filepathExampleInput).exists():
open(filepathExampleInput, 'a').close()
if __name__ == '__main__':
if len(sys.argv) > 1:
day = sys.argv[1]
else:
files = [Path(f).stem for f in os.listdir() if Path(f).is_file()]
day = max([int(f.replace('day', '')) for f in files if f.startswith('day')], default=0) + 1
filepath = os.path.join(f'day{day}.py')
filepathInput = os.path.join('Input', f'day{day}.txt')
filepathExampleInput = os.path.join('Input', f'day{day}_example.txt')
createFiles(filepath, filepathInput, filepathExampleInput, day) <file_sep>/2018/day7.py
input = """Step Y must be finished before step A can begin.
Step O must be finished before step C can begin.
Step P must be finished before step A can begin.
Step D must be finished before step N can begin.
Step T must be finished before step G can begin.
Step L must be finished before step M can begin.
Step X must be finished before step V can begin.
Step C must be finished before step R can begin.
Step G must be finished before step E can begin.
Step H must be finished before step N can begin.
Step Q must be finished before step B can begin.
Step S must be finished before step R can begin.
Step M must be finished before step F can begin.
Step N must be finished before step Z can begin.
Step E must be finished before step I can begin.
Step A must be finished before step R can begin.
Step Z must be finished before step F can begin.
Step K must be finished before step V can begin.
Step I must be finished before step J can begin.
Step R must be finished before step W can begin.
Step B must be finished before step J can begin.
Step W must be finished before step V can begin.
Step V must be finished before step F can begin.
Step U must be finished before step F can begin.
Step F must be finished before step J can begin.
Step X must be finished before step C can begin.
Step T must be finished before step Q can begin.
Step B must be finished before step F can begin.
Step Y must be finished before step L can begin.
Step P must be finished before step E can begin.
Step A must be finished before step J can begin.
Step S must be finished before step I can begin.
Step S must be finished before step A can begin.
Step K must be finished before step R can begin.
Step D must be finished before step C can begin.
Step R must be finished before step U can begin.
Step K must be finished before step U can begin.
Step D must be finished before step K can begin.
Step S must be finished before step M can begin.
Step D must be finished before step E can begin.
Step A must be finished before step K can begin.
Step G must be finished before step I can begin.
Step O must be finished before step M can begin.
Step U must be finished before step J can begin.
Step T must be finished before step S can begin.
Step C must be finished before step M can begin.
Step S must be finished before step J can begin.
Step N must be finished before step V can begin.
Step P must be finished before step N can begin.
Step D must be finished before step M can begin.
Step A must be finished before step B can begin.
Step Z must be finished before step R can begin.
Step T must be finished before step N can begin.
Step K must be finished before step J can begin.
Step N must be finished before step A can begin.
Step M must be finished before step R can begin.
Step E must be finished before step A can begin.
Step Y must be finished before step O can begin.
Step O must be finished before step B can begin.
Step O must be finished before step A can begin.
Step I must be finished before step V can begin.
Step M must be finished before step Z can begin.
Step D must be finished before step U can begin.
Step O must be finished before step S can begin.
Step Z must be finished before step W can begin.
Step M must be finished before step A can begin.
Step N must be finished before step E can begin.
Step M must be finished before step U can begin.
Step R must be finished before step J can begin.
Step W must be finished before step F can begin.
Step I must be finished before step U can begin.
Step E must be finished before step U can begin.
Step Y must be finished before step R can begin.
Step Z must be finished before step K can begin.
Step C must be finished before step F can begin.
Step B must be finished before step V can begin.
Step G must be finished before step B can begin.
Step O must be finished before step G can begin.
Step E must be finished before step Z can begin.
Step A must be finished before step V can begin.
Step Y must be finished before step Q can begin.
Step P must be finished before step D can begin.
Step X must be finished before step G can begin.
Step I must be finished before step W can begin.
Step M must be finished before step V can begin.
Step T must be finished before step M can begin.
Step G must be finished before step J can begin.
Step T must be finished before step I can begin.
Step H must be finished before step B can begin.
Step C must be finished before step E can begin.
Step Q must be finished before step V can begin.
Step H must be finished before step U can begin.
Step X must be finished before step K can begin.
Step D must be finished before step T can begin.
Step X must be finished before step W can begin.
Step P must be finished before step Z can begin.
Step C must be finished before step U can begin.
Step Y must be finished before step Z can begin.
Step L must be finished before step F can begin.
Step C must be finished before step J can begin.
Step T must be finished before step W can begin."""
result = []
import re
import string
def prepareData():
splitInput = input.split('\n')
dic = {}
for condition in splitInput:
match = re.search('\s([A-Z])\smust be finished before step ([A-Z])', condition)
letters = match.groups()
if letters[0] not in dic:
dic[letters[0]] = []
if letters[1] not in dic:
dic[letters[1]] = []
dic[letters[1]].append(letters[0])
return dic
def work(inWork, result):
for entry in inWork.copy():
inWork[entry] -= 1
if inWork[entry] == 0:
result.append(entry)
inWork.pop(entry)
return inWork
def doOne():
dic = prepareData()
ready = []
result = []
while (len(dic) > 0):
for key in dic.copy():
isReady = True
conditions = dic[key]
for condition in conditions:
if condition not in result:
isReady = False
break
if isReady:
ready.append(key)
dic.pop(key)
ready.sort()
ready.reverse()
result.append(ready.pop())
return result
def doTwo():
dic = prepareData()
matching = {}
count = 61
for x in string.ascii_uppercase:
matching[x] = count
count += 1
result = []
ready = []
inWork = {}
time = 0
while (True):
time += 1
for key in dic.copy():
isReady = True
conditions = dic[key]
for condition in conditions:
if condition not in result:
isReady = False
break
if isReady:
ready.append(key)
dic.pop(key)
ready.sort()
ready.reverse()
while len(inWork) < 5 and len(ready) > 0:
letter = ready.pop()
inWork[letter] = matching[letter]
inWork = work(inWork, result)
if len(inWork) == 0 and len(dic) == 0:
return time
result = doTwo()
print(result)<file_sep>/2020/day17.py
from HelperFunctions import inputsplit
from copy import deepcopy
def do1(puzzleInput):
space = prepareInput(puzzleInput, 3)
newSpace = changeState(space, 3)
return countActiveCubes(newSpace, 3)
def do2(puzzleInput):
space = prepareInput(puzzleInput, 4)
newSpace = changeState(space, 4)
return countActiveCubes(newSpace, 4)
def changeState(space, dimension):
newSpace = deepcopy(space)
for _ in range(6):
space = deepcopy(newSpace)
for x in range(1, len(space) - 1):
for y in range(1, len(space[x]) - 1):
for z in range(1, len(space[x][y]) - 1):
if dimension == 4:
for w in range(1, len(space[x][y][z]) - 1):
activeNeighbors = numberOfActiveNeighbors((x,y,z,w), space, dimension)
if space[x][y][z][w] == '#' and not (2 <= activeNeighbors <= 3):
newSpace[x][y][z][w] = '.'
elif space[x][y][z][w] == '.' and activeNeighbors == 3:
newSpace[x][y][z][w] = '#'
else:
activeNeighbors = numberOfActiveNeighbors((x,y,z), space, dimension)
if space[x][y][z] == '#' and not (2 <= activeNeighbors <= 3):
newSpace[x][y][z] = '.'
elif space[x][y][z] == '.' and activeNeighbors == 3:
newSpace[x][y][z] = '#'
return newSpace
def numberOfActiveNeighbors(position, space, dimension):
if dimension == 3:
x,y,z = position
else:
x,y,z,w = position
numberOfActiveNeighbors = 0
step = [-1, 0, 1]
for xStep in step:
for yStep in step:
for zStep in step:
if dimension == 4:
for wStep in step:
if xStep == 0 and yStep == 0 and zStep == 0 and wStep == 0:
continue
if space[x + xStep][y + yStep][z + zStep][w + wStep] == '#':
numberOfActiveNeighbors += 1
else:
if xStep == 0 and yStep == 0 and zStep == 0:
continue
if space[x + xStep][y + yStep][z + zStep] == '#':
numberOfActiveNeighbors += 1
return numberOfActiveNeighbors
def prepareInput(puzzleInput, dimension):
size = 31
if dimension == 3:
space = [[['.' for z in range(size)] for y in range(size)] for x in range(size)]
elif dimension == 4:
space = [[[['.' for w in range(size)] for z in range(size)] for y in range(size)] for x in range(size)]
w = int(size / 2)
z = int(size / 2)
x = int(size / 2 - int(len(puzzleInput) / 2))
for a in range(len(puzzleInput)):
y = int(size / 2 - int(len(puzzleInput[0]) / 2))
for b in range(len(puzzleInput[0])):
if dimension == 4:
space[x][y][z][w] = puzzleInput[a][b]
elif dimension == 3:
space[x][y][z] = puzzleInput[a][b]
y += 1
x += 1
return space
def countActiveCubes(space, dimension):
count = 0
for x in range(len(space)):
for y in range(len(space[x])):
if dimension == 4:
for z in range(len(space[x][y])):
count += space[x][y][z].count('#')
elif dimension == 3:
count += space[x][y].count('#')
return count
def do():
with open ('Input/day17.txt') as f:
strInput = f.read()
splitInput = inputsplit(strInput, '\n')
print(do1(splitInput))
print(do2(splitInput))
do()<file_sep>/2022/day21.py
from collections import namedtuple
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
NumberMonkey = namedtuple('NumberMonkey', ['name', 'number'])
OperationMonkey = namedtuple('OperationMonkey', ['name', 'operation', 'first', 'second'])
def add(first,second):
return first + second
def substract(first,second):
return first - second
def multiply(first,second):
return first * second
def divide(first,second):
return first / second
operations = {'+': add, '-': substract, '*': multiply, '/': divide}
def do1(splitInput):
monkeys = extractMonkeyInformation(splitInput)
return getNumber('root', monkeys)
def do2(splitInput):
monkeys = extractMonkeyInformation(splitInput)
i1,i2 = [0, 1000000000000]
monkeys['humn'] = NumberMonkey('humn', i1)
first1 = getNumber(monkeys['root'].first, monkeys)
monkeys['humn'] = NumberMonkey('humn', i2)
first2 = getNumber(monkeys['root'].first, monkeys)
second = getNumber(monkeys['root'].second, monkeys)
firstDiff = first1-first2
secondDiff = abs(second - first2)
missingDiff = secondDiff / firstDiff
return int(missingDiff * i2 + i2 + 1)
def extractMonkeyInformation(splitInput):
monkeys = {}
for line in splitInput:
match line.split(' '):
case [monkey, number]:
monkey = monkey[0:-1]
number = int(number)
monkeys[monkey] = NumberMonkey(monkey, number)
case [monkey, first, operation, second]:
monkey = monkey[0:-1]
monkeys[monkey] = OperationMonkey(monkey,operations[operation],first,second)
return monkeys
def getNumber(monkeyName, monkeys):
monkey = monkeys[monkeyName]
if type(monkey) is NumberMonkey:
return monkey.number
return monkey.operation(getNumber(monkey.first, monkeys), getNumber(monkey.second, monkeys))
def do():
strInput = readInputFile(21)
splitInput = strInput.split('\n')
print(do1(splitInput))
print(do2(splitInput))
print('done')
do()<file_sep>/2022/day3.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
def do1(splitInput):
prioritySum = 0
for rucksack in splitInput:
firstCompartment = rucksack[0:int(len(rucksack)/2)]
secondCompartment = rucksack[int(len(rucksack)/2):]
sameType = [x for x in firstCompartment if x in secondCompartment][0]
prioritySum += calculatePriorityValue(sameType)
return prioritySum
def do2(splitInput):
prioritySum = 0
groups = [splitInput[i:i+3] for i in range(0, len(splitInput), 3)]
for elf1,elf2,elf3 in groups:
sameType = [x for x in elf1 if x in elf2 and x in elf3][0]
prioritySum += calculatePriorityValue(sameType)
return prioritySum
def calculatePriorityValue(item):
if item.islower():
return ord(item) - ord('a') + 1
elif item.isupper():
return ord(item) - ord('A') + 27
def do():
strInput = readInputFile(3)
splitInput = strInput.split('\n')
print(do1(splitInput))
print(do2(splitInput))
print('done')
do()<file_sep>/2019/day4.py
rangeLow = 134792
rangeHigh = 675810
def do1():
result = []
for i in range(rangeLow, rangeHigh + 1):
if hasDouble(i) and doesNotDecrease(i):
result.append(i)
return result
def do2():
result = []
for i in range(rangeLow, rangeHigh + 1):
if hasOnlyOneDouble(i) and doesNotDecrease(i):
result.append(i)
return result
def hasOnlyOneDouble(i):
numbers = [int(x) for x in str(i)]
dic = {}
for number in numbers:
dic[number] = dic.get(number, 0) + 1
if 2 in dic.values():
return True
return False
def hasDouble(i):
numbers = [int(x) for x in str(i)]
for j in range(len(numbers) - 1):
if numbers[j] == numbers[j + 1]:
return True
return False
def doesNotDecrease(i):
numbers = [int(x) for x in str(i)]
for j in range(len(numbers) - 1):
if numbers[j+1] < numbers[j]:
return False
return True
#part1
print(len(do1()))
#part2
print(len(do2()))<file_sep>/2021/day11.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
def do1(splitInput):
octopuses = prepareInput(splitInput)
steps = 100
sumOfFlashes = 0
for _ in range(steps):
octopuses,flashes = flashOctopuses(octopuses)
sumOfFlashes += flashes
return sumOfFlashes
def do2(splitInput):
octopuses = prepareInput(splitInput)
steps = 0
flashes = 0
while flashes < len(splitInput) * len(splitInput[0]):
steps += 1
octopuses,flashes = flashOctopuses(octopuses)
return steps
def flashOctopuses(octopuses):
#increase energy level
energyOctopuses = []
for x in range(len(octopuses)):
energyOctopuses.append([y + 1 if y != -1 else -1 for y in octopuses[x] ])
flashes = 0
newFlashes = 1
flashed = []
while newFlashes != 0:
newFlashes = 0
for lineNumber,line in enumerate(energyOctopuses):
for position,octopus in enumerate(line):
if octopus == -1:
continue
elif octopus > 9:
flash(lineNumber, position, energyOctopuses, flashed)
newFlashes += 1
flashes += newFlashes
return energyOctopuses,flashes
def flash(line, position, octopuses, flashed):
if octopuses[line][position] < 10:
print('Why are we here?')
return
octopuses[line][position] = 0
flashed.append((line, position))
for lineDiff in [-1, 0, 1]:
for positionDiff in [-1, 0, 1]:
if lineDiff == 0 and positionDiff == 0:
continue
if octopuses[line + lineDiff][position + positionDiff] != -1 and (line + lineDiff, position + positionDiff) not in flashed:
octopuses[line + lineDiff][position + positionDiff] += 1
def prepareInput(splitInput):
octopuses = []
octopuses.append([-1] + [-1 for _ in range(len(splitInput[0]))] + [-1])
for line in splitInput:
octoLine = [-1] + convertToInt(line) + [-1]
octopuses.append(octoLine)
octopuses.append([-1 for _ in range(len(octopuses[0]))])
return octopuses
def do():
strInput = readInputFile(11)
splitInput = strInput.split('\n')
print(do1(splitInput))
print(do2(splitInput))
print('done')
do()<file_sep>/2022/day25.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
add = {'=':'-', '-':'0','0':'1','1':'2','2':'3'}
divide = {3:'=', 4:'-'}
def do1(splitInput):
sumOfNumbers = 0
for number in splitInput:
sumOfNumbers += convertToDecimal(number)
return convertToSnafu(sumOfNumbers)
def do2(splitInput):
return 'nothing to do'
def convertToDecimal(number):
decimal = 0
number = number[::-1]
for i in range(0,len(number)):
match number[i]:
case '=':
decimal -= 2*pow(5,i)
case '-':
decimal -= pow(5,i)
case num:
decimal += pow(5,i) * int(num)
return decimal
def convertToSnafu(number):
i = 0
while True:
if number < pow(5,i):
i = i-1
break
i += 1
result = ''
remaining = number
while remaining:
divided = int(remaining / (pow(5,i)))
if divided > 2:
if result:
result = result[0:-1] + add[result[-1]] + divide[divided]
result = updateResult(result)
else:
result = '1' + divide[divided]
else:
result = result + str(divided)
remaining = remaining % (pow(5,i))
i -= 1
for _ in range(i,-1,-1):
result += '0'
return result
def updateResult(result):
if '3' in result:
position = result.index('3')
if position == 0:
return '1=' + result[1:]
else:
result = result[0:position-1] + add[result[position-1]] + '=' + result[position+1:]
result = updateResult(result)
return result
def do():
strInput = readInputFile(25)
splitInput = strInput.split('\n')
print(do1(splitInput))
print(do2(splitInput))
print('done')
do()<file_sep>/2020/day14.py
from HelperFunctions import inputsplit
import re
def do1(puzzleInput):
memory = fillMemory(puzzleInput, updateValue)
return sum([x for x in memory.values()])
def do2(puzzleInput):
memory = fillMemory(puzzleInput, updateMemoryValue)
return sum([x for x in memory.values()])
def fillMemory(puzzleInput, updateFunction):
memory = {}
bitmask = 'XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX'
for line in puzzleInput:
match = re.match('mask = (.+)', line)
if match:
bitmask = match.groups()[0]
else:
match = re.match('mem\[([0-9]+)\] = ([0-9]+)', line)
if match:
position = int(match.groups()[0])
value = int(match.groups()[1])
updateFunction(bitmask, position, value, memory)
else:
print('could not match')
return memory
def updateMemoryValue(bitmask, position, value, memory):
memoryPositions = []
positionBinary = format(position, '036b')
memoryPositions.append(positionBinary)
for bit in range(len(bitmask)):
if bitmask[bit] == '1':
for position in range(len(memoryPositions)):
memoryPositions[position] = memoryPositions[position][0:bit] + '1' + memoryPositions[position][bit+1:]
elif bitmask[bit] == 'X':
for position in range(len(memoryPositions)):
memoryPositions[position] = memoryPositions[position][0:bit] + '0' + memoryPositions[position][bit+1:]
memoryPosition2 = memoryPositions[position][0:bit] + '1' + memoryPositions[position][bit+1:]
memoryPositions.append(memoryPosition2)
for memoryPosition in memoryPositions:
intMemoryPosition = int(memoryPosition, 2)
memory[intMemoryPosition] = value
def updateValue(bitmask, position, value, memory):
valueBinary = format(value, '036b')
for bit in range(len(bitmask)):
if bitmask[bit] == '1':
valueBinary = valueBinary[0:bit] + '1' + valueBinary[bit+1:]
elif bitmask[bit] == '0':
valueBinary = valueBinary[0:bit] + '0' + valueBinary[bit+1:]
intValue = int(valueBinary, 2)
memory[position] = intValue
def do():
with open ('Input/day14.txt') as f:
strInput = f.read()
splitInput = inputsplit(strInput, '\n')
print(do1(splitInput))
print(do2(splitInput))
do()<file_sep>/2018/day12.py
start = "#.##.##.##.##.......###..####..#....#...#.##...##.#.####...#..##..###...##.#..#.##.#.#.#.#..####..#"
transition = """..### => .
##..# => #
#..## => .
.#..# => .
#.##. => .
#.... => .
##... => #
#...# => .
###.# => #
##.## => .
....# => .
..##. => #
..#.. => .
##.#. => .
.##.# => #
#..#. => #
.##.. => #
###.. => #
.###. => #
##### => #
####. => .
.#.#. => .
...#. => #
#.### => .
.#... => #
.#### => .
#.#.# => #
...## => .
..... => .
.#.## => #
..#.# => #
#.#.. => #"""
addition = 600
import re
def prepareTransitions():
dic = {}
splitTransitions = transition.split('\n')
for entry in splitTransitions:
match = re.match('(.*) => (.)', entry)
pattern, result = match.groups()
dic[pattern] = result
return dic
def calculateSum(result):
totalSum = 0
for i in range(len(result)):
if result[i] == '#':
totalSum += i - addition
return totalSum
def do():
dic = prepareTransitions()
points = ''
for i in range(addition):
points += '.'
input = points + start + points
for i in range(20):
result = '..'
for index in range(2, len(input) - 2):
sub = input[index - 2: index + 3]
result += dic[sub]
input = result + '..'
sum = calculateSum(result)
return sum
print(do())
print(6900 * (50000000000 / 100 - 2) + 16068)<file_sep>/2020/day25.py
from HelperFunctions import inputsplit
def do1(puzzleInput):
cardPublic = int(puzzleInput[0])
doorPublic = int(puzzleInput[1])
loopSizeCard = getLoopSize(cardPublic, 7)
return calculateEncryptionKey(loopSizeCard, doorPublic)
def do2(puzzleInput):
return 'done'
def getLoopSize(publicKey, subjectNumber):
value = 1
loop = 0
while value != publicKey:
loop += 1
value = value * subjectNumber
value = value % 20201227
return loop
def calculateEncryptionKey(loopSize, subjectNumber):
value = 1
for _ in range(loopSize):
value = value * subjectNumber
value = value % 20201227
return value
def do():
with open ('Input/day25.txt') as f:
strInput = f.read()
puzzleInput = strInput.split('\n')
print(do1(puzzleInput))
print(do2(puzzleInput))
do()<file_sep>/2021/HelperFunctions.py
def readInputFile(day):
with open (f'Input/day{day}.txt') as f:
strInput = f.read()
return strInput
def readExampleInput(day):
with open(f'Input/day{day}_example.txt') as f:
strInput = f.read()
return strInput
def convertToInt(input):
numbers = []
for stringNumber in input:
numbers.append(int(stringNumber))
return numbers<file_sep>/2020/day4.py
from HelperFunctions import inputsplit
import re
def do1(puzzleInput):
passportKeys = ['byr', 'iyr', 'eyr', 'hgt', 'hcl', 'ecl', 'pid']
passportData = extractPassportData(puzzleInput)
numberOfValidPassports = 0
for passport in passportData:
if all (x in passport.keys() for x in passportKeys):
numberOfValidPassports += 1
return numberOfValidPassports
def do2(puzzleInput):
passportData = extractPassportData(puzzleInput)
passportKeys = ['byr', 'iyr', 'eyr', 'hgt', 'hcl', 'ecl', 'pid']
numberOfValidPassports = 0
for passport in passportData:
if all (x in passport.keys() for x in passportKeys):
if (checkBirthYear(passport) and checkIssueYear(passport) and checkExpirationYear(passport) and checkHeight(passport) and checkHairColor(passport) and checkPassportID(passport) and checkEyeColor(passport)):
numberOfValidPassports += 1
return numberOfValidPassports
def checkBirthYear(passport):
if passport['byr'].isnumeric and 1920 <= int(passport['byr']) <= 2002:
return True
return False
def checkIssueYear(passport):
if passport['iyr'].isnumeric and 2010 <= int(passport['iyr']) <= 2020:
return True
return False
def checkExpirationYear(passport):
if passport['eyr'].isnumeric and 2020 <= int(passport['eyr']) <= 2030:
return True
return False
def checkHeight(passport):
match = re.fullmatch('([0-9]+)(\D+)', passport['hgt'])
if match and match.groups()[1] == 'cm' and 150 <= int(match.groups()[0]) <= 193:
return True
if match and match.groups()[1] == 'in' and 59 <= int(match.groups()[0]) <= 76:
return True
return False
def checkHairColor(passport):
match = re.fullmatch('\#[0-9|a-f]{6}', passport['hcl'])
return match
def checkPassportID(passport):
match = re.fullmatch('[0-9]{9}', passport['pid'])
return match
def checkEyeColor(passport):
validEyeColors = ['amb', 'blu', 'brn', 'gry', 'grn', 'hzl', 'oth']
return passport['ecl'] in validEyeColors
def extractPassportData(puzzleInput):
resultList = []
for passportData in puzzleInput:
passport = {}
match = re.findall('([a-z]+):([\#a-zA-Z0-9]+)[\s]?', passportData)
for entries in match:
passport[entries[0]] = entries[1]
resultList.append(passport)
return resultList
def do():
with open ('Input/day4.txt') as f:
strInput = f.read()
splitInput = inputsplit(strInput, '\n\n')
print(do1(splitInput))
print(do2(splitInput))
do()<file_sep>/2021/day7.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
def do1(crabs):
fuelSum = 0
minFuelSum = 10000000
for i in range(min(crabs), max(crabs) + 1):
fuelSum = 0
for crab in crabs:
fuel = abs(i - crab)
fuelSum += fuel
minFuelSum = min(minFuelSum, fuelSum)
return minFuelSum
def do2(crabs):
maxCrabs = max(crabs) + 1
crabRange = range(min(crabs), maxCrabs)
steps = [i for i in range(1, maxCrabs)]
fuelNeeded = [sum(steps[0:i]) for i in range(1, maxCrabs)]
minFuelSum = 10000000000
for i in crabRange:
fuelSum = 0
for crab in crabs:
stepsNeeded = abs(i - crab)
fuel = fuelNeeded[stepsNeeded - 1]
fuelSum += fuel
minFuelSum = min(minFuelSum, fuelSum)
return minFuelSum
def do():
strInput = readInputFile(7)
splitInput = strInput.split(',')
intInput = convertToInt(splitInput)
print(do1(intInput))
print(do2(intInput))
print('done')
do()<file_sep>/2018/day5.py
original="""<KEY>"""
def do(input):
characters = 'abcdefghijklmnopqrstuvwxyz'
dic = {}
for character in characters:
input = original
changed = True
input = input.replace(character, '')
input = input.replace(character.upper(), '')
while(changed):
changed = False
i = 0
while(i < len(input) - 2):
chr1 = input[i]
chr2 = input[i + 1]
if chr1.islower() and chr2.isupper():
if chr1.upper() == chr2:
input = input[:i] + input[i+2:]
changed = True
if i > 0:
i -= 1
elif chr1.isupper() and chr2.islower():
if chr1.lower() == chr2:
input = input[:i] + input[i+2:]
changed = True
if i > 0:
i -= 1
i += 1
dic[character] = len(input)
return dic
result = do(input)
print(min(result.values()))
<file_sep>/2021/day5.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
import re
from collections import defaultdict
def do1(splitInput):
points = defaultdict(lambda: 0)
parsedLines = parseLines(splitInput)
for line in parsedLines:
x1,x2,y1,y2 = line
addPoints(x1, x2, y1, y2, points, False)
multiples = findMultiples(points)
return len(multiples)
def do2(splitInput):
points = defaultdict(lambda: 0)
parsedLines = parseLines(splitInput)
for line in parsedLines:
x1,x2,y1,y2 = line
addPoints(x1, x2, y1, y2, points, True)
multiples = findMultiples(points)
return len(multiples)
def parseLines(splitInput):
parsedLines = []
for line in splitInput:
match = re.fullmatch('(?P<x1>[0-9]+),(?P<y1>[0-9]+) -> (?P<x2>[0-9]+),(?P<y2>[0-9]+)', line)
if match:
x1 = int(match.group('x1'))
x2 = int(match.group('x2'))
y1 = int(match.group('y1'))
y2 = int(match.group('y2'))
parsedLines.append((x1,x2,y1,y2))
return parsedLines
def addPoints(x1,x2,y1,y2, points, diagonalsAllowed):
x = sorted([x1,x2])
y = sorted([y1,y2])
if x1 == x2:
for i in range(y[0], y[1] + 1):
points[(x1, i)] += 1
elif y1 == y2:
for i in range(x[0], x[1] + 1):
points[(i, y1)] += 1
else:
if not diagonalsAllowed:
return
else:
factorX = 1
factorY = 1
if x1 > x2:
factorX = -1
if y1 > y2:
factorY = -1
for i in range(x[1] - x[0] + 1):
points[(x1 + factorX * i, y1 + factorY * i)] += 1
def findMultiples(points):
multiples = [x for x in points.keys() if points[x] > 1]
return multiples
def do():
strInput = readInputFile(5)
splitInput = strInput.split('\n')
print(do1(splitInput))
print(do2(splitInput))
print('done')
do()<file_sep>/2018/day4.py
input = """[1518-03-25 00:01] Guard #743 begins shift
[1518-09-15 00:34] falls asleep
[1518-10-11 00:27] wakes up
[1518-04-30 00:56] falls asleep
[1518-05-27 00:33] falls asleep
[1518-11-07 00:52] wakes up
[1518-09-04 00:47] wakes up
[1518-05-29 00:44] wakes up
[1518-06-10 00:41] falls asleep
[1518-09-03 00:21] wakes up
[1518-06-16 00:59] wakes up
[1518-03-04 00:52] wakes up
[1518-07-13 00:58] wakes up
[1518-07-31 00:05] falls asleep
[1518-10-15 00:55] falls asleep
[1518-06-15 23:46] Guard #2113 begins shift
[1518-08-07 00:00] Guard #1439 begins shift
[1518-10-28 00:27] falls asleep
[1518-08-17 00:53] wakes up
[1518-05-20 00:07] falls asleep
[1518-06-23 00:30] falls asleep
[1518-09-08 00:43] wakes up
[1518-08-30 00:00] Guard #463 begins shift
[1518-05-27 00:18] falls asleep
[1518-10-26 00:06] falls asleep
[1518-11-16 00:54] wakes up
[1518-04-15 00:17] wakes up
[1518-04-10 23:53] Guard #463 begins shift
[1518-11-23 00:46] wakes up
[1518-03-19 00:57] wakes up
[1518-08-19 00:48] falls asleep
[1518-03-05 00:44] wakes up
[1518-06-30 00:03] falls asleep
[1518-05-19 00:49] wakes up
[1518-10-30 00:31] falls asleep
[1518-02-25 00:28] wakes up
[1518-04-05 00:48] falls asleep
[1518-04-06 23:56] Guard #151 begins shift
[1518-09-24 00:27] falls asleep
[1518-10-12 00:19] falls asleep
[1518-07-22 00:08] wakes up
[1518-08-28 00:03] Guard #3041 begins shift
[1518-08-09 00:03] falls asleep
[1518-04-25 00:11] falls asleep
[1518-05-21 23:57] Guard #907 begins shift
[1518-11-04 00:04] falls asleep
[1518-08-08 23:48] Guard #151 begins shift
[1518-10-06 00:27] falls asleep
[1518-10-31 00:48] wakes up
[1518-09-08 00:53] falls asleep
[1518-10-25 00:02] Guard #643 begins shift
[1518-06-12 00:58] wakes up
[1518-03-26 23:57] Guard #1439 begins shift
[1518-06-07 00:16] falls asleep
[1518-08-18 00:34] wakes up
[1518-06-06 00:50] wakes up
[1518-10-16 00:04] falls asleep
[1518-03-26 00:01] wakes up
[1518-08-20 00:51] falls asleep
[1518-10-15 00:24] falls asleep
[1518-08-30 00:54] wakes up
[1518-09-23 23:56] Guard #251 begins shift
[1518-05-06 00:00] Guard #487 begins shift
[1518-08-06 00:22] wakes up
[1518-06-17 00:58] wakes up
[1518-03-12 00:41] wakes up
[1518-10-10 23:47] Guard #643 begins shift
[1518-06-24 00:19] falls asleep
[1518-06-24 00:39] falls asleep
[1518-08-07 00:45] wakes up
[1518-06-26 00:15] falls asleep
[1518-06-18 00:40] falls asleep
[1518-11-16 23:57] Guard #3323 begins shift
[1518-11-10 00:22] wakes up
[1518-10-27 00:01] Guard #1297 begins shift
[1518-04-01 00:43] falls asleep
[1518-08-05 23:59] Guard #251 begins shift
[1518-07-10 00:26] falls asleep
[1518-09-26 00:45] falls asleep
[1518-10-30 00:44] wakes up
[1518-10-20 00:01] Guard #3209 begins shift
[1518-04-21 00:54] wakes up
[1518-05-28 23:50] Guard #1297 begins shift
[1518-08-11 00:48] wakes up
[1518-06-16 00:29] wakes up
[1518-05-14 00:37] falls asleep
[1518-03-12 23:57] Guard #463 begins shift
[1518-04-24 00:45] wakes up
[1518-07-17 00:34] falls asleep
[1518-06-01 00:52] falls asleep
[1518-08-26 00:01] Guard #1297 begins shift
[1518-10-10 00:50] falls asleep
[1518-09-15 00:29] wakes up
[1518-07-23 23:58] Guard #463 begins shift
[1518-11-21 00:02] Guard #3041 begins shift
[1518-07-02 00:44] falls asleep
[1518-09-13 00:31] falls asleep
[1518-07-12 00:50] wakes up
[1518-04-09 00:53] wakes up
[1518-11-12 00:08] wakes up
[1518-04-11 00:00] falls asleep
[1518-03-17 00:46] wakes up
[1518-10-09 23:57] Guard #1439 begins shift
[1518-05-25 23:58] Guard #151 begins shift
[1518-04-23 00:03] Guard #263 begins shift
[1518-05-13 00:00] Guard #1297 begins shift
[1518-05-01 00:31] wakes up
[1518-04-02 00:27] wakes up
[1518-11-13 00:47] wakes up
[1518-02-28 23:56] Guard #3323 begins shift
[1518-08-16 00:45] wakes up
[1518-07-11 00:54] wakes up
[1518-07-26 00:17] wakes up
[1518-09-30 00:52] falls asleep
[1518-05-13 23:57] Guard #151 begins shift
[1518-05-05 00:52] wakes up
[1518-07-01 00:29] falls asleep
[1518-09-08 00:17] falls asleep
[1518-04-28 23:56] Guard #487 begins shift
[1518-04-25 00:03] Guard #263 begins shift
[1518-06-06 00:03] Guard #1439 begins shift
[1518-06-25 00:33] wakes up
[1518-07-05 00:32] wakes up
[1518-05-12 00:00] falls asleep
[1518-08-13 23:47] Guard #487 begins shift
[1518-08-22 00:29] falls asleep
[1518-03-23 23:57] Guard #1439 begins shift
[1518-08-09 23:48] Guard #3517 begins shift
[1518-08-31 00:40] wakes up
[1518-11-14 00:03] Guard #487 begins shift
[1518-05-12 00:33] wakes up
[1518-11-19 00:00] Guard #1019 begins shift
[1518-05-04 00:59] wakes up
[1518-06-12 00:47] falls asleep
[1518-03-23 00:00] Guard #263 begins shift
[1518-11-04 00:23] falls asleep
[1518-06-13 00:01] falls asleep
[1518-07-15 00:04] Guard #971 begins shift
[1518-07-19 00:25] falls asleep
[1518-04-26 00:48] falls asleep
[1518-06-30 00:29] wakes up
[1518-08-21 00:52] wakes up
[1518-09-09 23:59] Guard #3517 begins shift
[1518-03-22 00:00] Guard #3323 begins shift
[1518-08-27 00:04] Guard #251 begins shift
[1518-09-04 00:52] falls asleep
[1518-08-27 00:09] falls asleep
[1518-07-27 00:00] Guard #1439 begins shift
[1518-09-20 00:55] wakes up
[1518-09-14 00:37] wakes up
[1518-03-07 23:57] Guard #1439 begins shift
[1518-04-10 00:27] falls asleep
[1518-07-02 00:59] wakes up
[1518-04-21 00:51] falls asleep
[1518-04-12 00:23] falls asleep
[1518-08-17 00:06] falls asleep
[1518-02-22 00:48] wakes up
[1518-10-01 00:50] wakes up
[1518-02-21 00:00] Guard #1439 begins shift
[1518-10-30 00:03] Guard #509 begins shift
[1518-08-08 00:21] falls asleep
[1518-10-24 00:01] Guard #1297 begins shift
[1518-04-21 00:04] falls asleep
[1518-05-10 00:39] wakes up
[1518-05-10 00:00] Guard #3323 begins shift
[1518-03-10 00:50] wakes up
[1518-07-22 00:06] falls asleep
[1518-07-10 00:54] wakes up
[1518-11-06 00:22] falls asleep
[1518-04-05 00:54] wakes up
[1518-05-14 00:52] falls asleep
[1518-09-13 00:46] wakes up
[1518-07-15 23:59] Guard #151 begins shift
[1518-06-01 00:41] falls asleep
[1518-04-24 00:38] falls asleep
[1518-07-20 00:57] wakes up
[1518-03-30 00:59] wakes up
[1518-08-04 00:20] wakes up
[1518-09-26 00:10] falls asleep
[1518-03-30 00:02] Guard #3469 begins shift
[1518-07-09 00:52] wakes up
[1518-04-23 00:21] falls asleep
[1518-05-01 00:44] wakes up
[1518-04-04 23:58] Guard #3323 begins shift
[1518-08-07 00:25] falls asleep
[1518-06-09 23:58] Guard #3209 begins shift
[1518-07-09 00:48] falls asleep
[1518-08-11 00:47] falls asleep
[1518-03-09 00:07] falls asleep
[1518-06-29 00:41] wakes up
[1518-05-27 23:58] Guard #743 begins shift
[1518-05-19 00:43] falls asleep
[1518-03-17 00:33] falls asleep
[1518-07-12 00:49] falls asleep
[1518-11-03 00:15] wakes up
[1518-06-05 00:57] wakes up
[1518-11-07 00:24] falls asleep
[1518-07-06 00:12] falls asleep
[1518-05-26 23:59] Guard #907 begins shift
[1518-06-01 00:28] wakes up
[1518-11-09 00:04] falls asleep
[1518-06-27 00:49] wakes up
[1518-03-29 00:42] wakes up
[1518-05-21 00:47] falls asleep
[1518-08-04 00:47] wakes up
[1518-07-18 00:23] wakes up
[1518-09-07 23:56] Guard #463 begins shift
[1518-09-10 23:51] Guard #1019 begins shift
[1518-09-29 00:49] wakes up
[1518-06-15 00:57] wakes up
[1518-04-04 00:31] falls asleep
[1518-08-31 00:49] wakes up
[1518-10-23 00:59] wakes up
[1518-05-21 00:02] falls asleep
[1518-07-02 00:36] falls asleep
[1518-06-29 23:51] Guard #3517 begins shift
[1518-07-25 00:47] wakes up
[1518-11-15 00:39] wakes up
[1518-11-13 00:57] falls asleep
[1518-09-26 23:59] Guard #463 begins shift
[1518-03-10 00:22] wakes up
[1518-08-10 00:56] wakes up
[1518-07-28 00:31] falls asleep
[1518-10-12 00:43] wakes up
[1518-05-27 00:44] wakes up
[1518-07-28 00:32] wakes up
[1518-04-27 00:54] wakes up
[1518-08-12 00:39] falls asleep
[1518-04-03 00:38] wakes up
[1518-04-03 00:11] falls asleep
[1518-08-24 00:57] wakes up
[1518-05-15 00:52] wakes up
[1518-10-22 00:56] wakes up
[1518-04-24 00:00] Guard #509 begins shift
[1518-03-26 00:00] falls asleep
[1518-09-30 23:58] Guard #1297 begins shift
[1518-07-16 00:53] wakes up
[1518-05-23 00:15] falls asleep
[1518-11-13 00:59] wakes up
[1518-02-27 00:53] wakes up
[1518-08-19 00:42] wakes up
[1518-04-19 00:03] Guard #3209 begins shift
[1518-11-03 00:14] falls asleep
[1518-09-16 00:44] falls asleep
[1518-03-21 00:11] falls asleep
[1518-08-14 00:17] wakes up
[1518-09-04 00:35] falls asleep
[1518-10-14 00:41] wakes up
[1518-06-17 00:36] falls asleep
[1518-08-04 00:16] falls asleep
[1518-06-13 00:52] wakes up
[1518-03-07 00:02] Guard #251 begins shift
[1518-05-10 00:09] falls asleep
[1518-03-14 00:04] Guard #1439 begins shift
[1518-07-24 23:56] Guard #463 begins shift
[1518-11-01 00:25] falls asleep
[1518-06-23 00:44] wakes up
[1518-11-17 00:12] wakes up
[1518-06-13 00:22] falls asleep
[1518-08-01 00:47] falls asleep
[1518-04-17 00:11] falls asleep
[1518-09-12 00:01] falls asleep
[1518-07-05 23:57] Guard #3323 begins shift
[1518-03-16 00:57] falls asleep
[1518-05-26 00:08] falls asleep
[1518-04-09 00:57] falls asleep
[1518-11-14 00:09] falls asleep
[1518-11-10 00:00] Guard #1019 begins shift
[1518-09-08 00:41] falls asleep
[1518-03-30 00:41] falls asleep
[1518-10-22 00:43] wakes up
[1518-06-17 00:56] falls asleep
[1518-08-19 23:56] Guard #907 begins shift
[1518-03-01 00:39] wakes up
[1518-06-16 00:01] falls asleep
[1518-07-07 00:48] wakes up
[1518-07-29 23:50] Guard #907 begins shift
[1518-06-28 00:56] wakes up
[1518-11-22 00:44] wakes up
[1518-09-26 00:54] wakes up
[1518-04-08 00:01] Guard #1307 begins shift
[1518-02-23 00:58] wakes up
[1518-06-02 00:08] falls asleep
[1518-10-17 00:30] wakes up
[1518-11-02 00:56] wakes up
[1518-06-24 00:23] wakes up
[1518-05-03 00:43] wakes up
[1518-05-03 00:25] falls asleep
[1518-03-03 00:30] falls asleep
[1518-10-27 00:33] falls asleep
[1518-10-29 00:30] falls asleep
[1518-11-06 00:51] wakes up
[1518-04-21 00:28] wakes up
[1518-04-23 00:43] wakes up
[1518-09-04 00:00] Guard #1307 begins shift
[1518-06-22 00:45] wakes up
[1518-05-17 00:58] wakes up
[1518-05-05 00:44] wakes up
[1518-07-25 00:22] falls asleep
[1518-11-11 00:11] falls asleep
[1518-04-17 00:03] Guard #947 begins shift
[1518-11-19 00:08] falls asleep
[1518-09-03 00:43] wakes up
[1518-08-26 00:31] falls asleep
[1518-05-05 00:03] falls asleep
[1518-08-19 00:58] wakes up
[1518-02-28 00:11] falls asleep
[1518-08-27 00:48] falls asleep
[1518-09-08 00:56] wakes up
[1518-11-14 00:56] wakes up
[1518-11-12 23:56] Guard #1307 begins shift
[1518-04-01 00:51] falls asleep
[1518-09-11 00:19] falls asleep
[1518-08-15 00:13] falls asleep
[1518-08-03 23:57] Guard #3209 begins shift
[1518-11-11 00:04] Guard #947 begins shift
[1518-10-21 23:54] Guard #1033 begins shift
[1518-04-01 00:03] Guard #251 begins shift
[1518-11-08 23:51] Guard #3323 begins shift
[1518-06-11 23:56] Guard #3469 begins shift
[1518-10-12 23:56] Guard #1307 begins shift
[1518-05-04 00:28] falls asleep
[1518-08-03 00:18] wakes up
[1518-08-14 00:25] falls asleep
[1518-11-13 00:34] falls asleep
[1518-03-18 00:20] falls asleep
[1518-11-03 00:44] falls asleep
[1518-10-03 23:57] Guard #1033 begins shift
[1518-09-03 00:07] falls asleep
[1518-10-11 00:05] falls asleep
[1518-11-17 00:17] falls asleep
[1518-07-16 00:15] falls asleep
[1518-03-22 00:37] falls asleep
[1518-11-05 23:50] Guard #3517 begins shift
[1518-08-05 00:58] wakes up
[1518-11-05 00:55] wakes up
[1518-04-13 00:57] wakes up
[1518-07-23 00:09] falls asleep
[1518-09-12 00:51] wakes up
[1518-09-10 00:58] wakes up
[1518-07-19 00:57] wakes up
[1518-07-11 00:13] falls asleep
[1518-09-21 23:57] Guard #971 begins shift
[1518-04-08 00:43] falls asleep
[1518-08-22 00:39] wakes up
[1518-02-21 00:26] falls asleep
[1518-05-07 00:55] wakes up
[1518-11-16 00:03] Guard #509 begins shift
[1518-10-09 00:03] Guard #1439 begins shift
[1518-05-20 23:51] Guard #743 begins shift
[1518-06-05 00:45] falls asleep
[1518-04-18 00:01] falls asleep
[1518-04-08 00:20] falls asleep
[1518-08-08 00:03] Guard #3517 begins shift
[1518-09-03 00:31] falls asleep
[1518-10-15 00:59] wakes up
[1518-09-14 00:03] Guard #643 begins shift
[1518-08-18 00:21] falls asleep
[1518-09-05 00:43] wakes up
[1518-02-25 00:04] Guard #487 begins shift
[1518-04-04 00:51] wakes up
[1518-03-02 00:23] falls asleep
[1518-11-13 00:52] falls asleep
[1518-04-14 00:00] Guard #743 begins shift
[1518-05-16 00:43] wakes up
[1518-10-14 00:01] Guard #1297 begins shift
[1518-05-21 00:57] falls asleep
[1518-10-02 23:56] Guard #1019 begins shift
[1518-02-24 00:39] wakes up
[1518-11-11 00:56] wakes up
[1518-08-24 00:53] falls asleep
[1518-04-25 23:53] Guard #643 begins shift
[1518-09-09 00:27] wakes up
[1518-05-24 00:00] Guard #1019 begins shift
[1518-03-29 00:40] falls asleep
[1518-07-03 00:58] wakes up
[1518-05-30 00:53] wakes up
[1518-05-01 00:41] falls asleep
[1518-07-02 00:41] wakes up
[1518-09-05 00:02] Guard #1439 begins shift
[1518-06-20 00:29] falls asleep
[1518-09-12 00:34] wakes up
[1518-09-27 00:53] wakes up
[1518-08-10 00:34] falls asleep
[1518-07-04 00:00] Guard #643 begins shift
[1518-02-22 00:03] Guard #3517 begins shift
[1518-06-20 00:45] wakes up
[1518-11-01 23:50] Guard #947 begins shift
[1518-05-09 00:22] wakes up
[1518-04-13 00:56] falls asleep
[1518-08-11 00:16] falls asleep
[1518-07-02 00:57] falls asleep
[1518-07-02 00:45] wakes up
[1518-03-23 00:45] falls asleep
[1518-10-16 00:47] wakes up
[1518-04-26 00:05] falls asleep
[1518-08-14 23:56] Guard #3469 begins shift
[1518-09-16 23:59] Guard #509 begins shift
[1518-08-29 00:09] falls asleep
[1518-11-10 00:58] wakes up
[1518-09-30 00:55] wakes up
[1518-09-15 00:10] falls asleep
[1518-10-06 00:57] wakes up
[1518-04-12 00:43] wakes up
[1518-07-22 00:50] wakes up
[1518-05-29 23:50] Guard #263 begins shift
[1518-06-08 00:58] wakes up
[1518-05-02 00:44] wakes up
[1518-05-23 00:40] wakes up
[1518-04-14 23:58] Guard #3517 begins shift
[1518-11-08 00:57] falls asleep
[1518-09-10 00:51] falls asleep
[1518-09-26 00:03] Guard #151 begins shift
[1518-08-25 00:02] Guard #1307 begins shift
[1518-11-09 00:26] wakes up
[1518-03-25 00:52] wakes up
[1518-03-06 00:56] wakes up
[1518-06-09 00:48] falls asleep
[1518-04-14 00:53] wakes up
[1518-09-16 00:48] wakes up
[1518-09-07 00:53] wakes up
[1518-09-25 00:47] falls asleep
[1518-09-11 23:51] Guard #3323 begins shift
[1518-11-15 00:24] wakes up
[1518-05-02 00:20] falls asleep
[1518-03-23 00:57] wakes up
[1518-06-11 00:08] falls asleep
[1518-03-11 00:03] falls asleep
[1518-04-12 00:56] wakes up
[1518-05-11 00:56] wakes up
[1518-08-21 00:02] Guard #151 begins shift
[1518-03-12 00:04] Guard #509 begins shift
[1518-07-24 00:21] wakes up
[1518-04-03 00:02] Guard #3209 begins shift
[1518-05-24 23:57] Guard #487 begins shift
[1518-04-10 00:04] falls asleep
[1518-04-18 00:48] falls asleep
[1518-05-17 00:00] Guard #1297 begins shift
[1518-07-29 00:14] falls asleep
[1518-07-09 00:04] Guard #2113 begins shift
[1518-11-15 00:01] Guard #1297 begins shift
[1518-03-02 00:55] wakes up
[1518-06-17 00:00] Guard #1307 begins shift
[1518-06-28 00:50] falls asleep
[1518-07-03 00:50] falls asleep
[1518-03-27 00:56] wakes up
[1518-08-23 00:00] falls asleep
[1518-08-27 00:44] wakes up
[1518-03-18 00:57] wakes up
[1518-09-24 00:52] wakes up
[1518-09-27 00:09] falls asleep
[1518-05-26 00:49] wakes up
[1518-10-07 00:33] falls asleep
[1518-08-14 00:00] falls asleep
[1518-07-26 00:08] falls asleep
[1518-07-14 00:15] falls asleep
[1518-09-19 00:53] wakes up
[1518-10-26 00:40] wakes up
[1518-03-26 00:29] falls asleep
[1518-10-28 23:56] Guard #487 begins shift
[1518-09-21 00:58] wakes up
[1518-07-01 00:57] wakes up
[1518-09-04 00:55] wakes up
[1518-02-21 00:27] wakes up
[1518-02-25 00:58] wakes up
[1518-03-28 00:44] falls asleep
[1518-08-24 00:10] wakes up
[1518-09-02 00:57] wakes up
[1518-06-13 00:06] wakes up
[1518-04-16 00:00] Guard #971 begins shift
[1518-09-07 00:17] falls asleep
[1518-11-14 00:44] falls asleep
[1518-06-13 23:58] Guard #3041 begins shift
[1518-03-30 23:59] Guard #643 begins shift
[1518-11-10 00:07] falls asleep
[1518-08-01 00:57] wakes up
[1518-09-06 00:45] wakes up
[1518-07-01 00:41] falls asleep
[1518-04-23 00:57] falls asleep
[1518-10-19 00:15] falls asleep
[1518-07-24 00:11] falls asleep
[1518-09-15 23:59] Guard #487 begins shift
[1518-09-01 00:55] wakes up
[1518-11-20 00:14] falls asleep
[1518-09-12 23:56] Guard #509 begins shift
[1518-03-06 00:19] falls asleep
[1518-07-01 00:02] Guard #463 begins shift
[1518-10-15 00:47] wakes up
[1518-11-18 00:50] wakes up
[1518-09-30 00:15] falls asleep
[1518-06-19 00:39] wakes up
[1518-06-05 00:07] falls asleep
[1518-08-02 00:00] Guard #3517 begins shift
[1518-06-18 23:59] Guard #1297 begins shift
[1518-09-11 00:04] falls asleep
[1518-10-06 00:00] Guard #487 begins shift
[1518-04-09 00:58] wakes up
[1518-05-28 00:07] falls asleep
[1518-05-27 00:30] wakes up
[1518-11-07 00:03] Guard #643 begins shift
[1518-11-09 00:59] wakes up
[1518-08-31 00:35] falls asleep
[1518-04-29 00:09] falls asleep
[1518-03-10 00:37] falls asleep
[1518-09-25 00:54] wakes up
[1518-10-05 00:26] wakes up
[1518-06-27 00:25] falls asleep
[1518-07-08 00:00] Guard #251 begins shift
[1518-08-16 00:00] Guard #1439 begins shift
[1518-11-19 00:48] wakes up
[1518-05-22 00:21] falls asleep
[1518-11-21 23:58] Guard #509 begins shift
[1518-11-22 23:46] Guard #1297 begins shift
[1518-11-08 00:51] wakes up
[1518-04-20 23:50] Guard #1033 begins shift
[1518-03-19 00:54] falls asleep
[1518-08-02 00:39] falls asleep
[1518-08-21 00:18] falls asleep
[1518-05-31 23:57] Guard #509 begins shift
[1518-09-22 23:48] Guard #907 begins shift
[1518-10-20 23:56] Guard #947 begins shift
[1518-08-25 00:28] falls asleep
[1518-05-26 00:20] wakes up
[1518-11-08 00:45] falls asleep
[1518-10-03 00:40] wakes up
[1518-10-21 00:36] falls asleep
[1518-04-27 23:58] Guard #3041 begins shift
[1518-09-28 00:21] falls asleep
[1518-09-17 00:21] falls asleep
[1518-11-20 00:50] wakes up
[1518-05-10 00:28] falls asleep
[1518-08-05 00:32] falls asleep
[1518-10-02 00:00] Guard #263 begins shift
[1518-09-18 00:41] wakes up
[1518-06-05 00:03] Guard #263 begins shift
[1518-02-25 00:10] falls asleep
[1518-10-21 00:53] falls asleep
[1518-10-14 00:06] falls asleep
[1518-03-21 00:58] wakes up
[1518-03-05 00:18] falls asleep
[1518-03-15 00:57] wakes up
[1518-06-06 00:41] falls asleep
[1518-03-16 00:35] wakes up
[1518-10-22 00:54] falls asleep
[1518-07-04 00:25] falls asleep
[1518-09-23 00:27] wakes up
[1518-02-22 00:25] falls asleep
[1518-11-18 00:22] falls asleep
[1518-11-11 23:48] Guard #743 begins shift
[1518-04-04 00:01] Guard #643 begins shift
[1518-08-14 00:46] wakes up
[1518-08-02 00:51] wakes up
[1518-11-16 00:10] falls asleep
[1518-04-13 00:19] falls asleep
[1518-04-11 00:08] wakes up
[1518-06-21 00:08] falls asleep
[1518-11-22 00:55] wakes up
[1518-03-26 00:54] wakes up
[1518-09-18 00:59] wakes up
[1518-03-07 00:15] falls asleep
[1518-08-01 00:55] falls asleep
[1518-11-03 00:02] Guard #1307 begins shift
[1518-05-03 00:00] Guard #251 begins shift
[1518-04-02 00:00] Guard #251 begins shift
[1518-10-21 00:55] wakes up
[1518-06-03 23:47] Guard #1019 begins shift
[1518-05-08 00:23] falls asleep
[1518-02-23 00:42] falls asleep
[1518-06-09 00:59] wakes up
[1518-05-08 23:52] Guard #947 begins shift
[1518-09-18 00:21] falls asleep
[1518-03-15 23:56] Guard #3323 begins shift
[1518-05-25 00:39] falls asleep
[1518-06-30 00:32] falls asleep
[1518-06-08 00:43] wakes up
[1518-07-01 00:49] wakes up
[1518-10-03 00:18] falls asleep
[1518-10-15 00:04] Guard #151 begins shift
[1518-07-18 00:09] falls asleep
[1518-05-04 23:50] Guard #263 begins shift
[1518-09-20 23:56] Guard #3517 begins shift
[1518-07-11 00:01] Guard #643 begins shift
[1518-09-12 00:13] wakes up
[1518-03-22 00:44] wakes up
[1518-10-01 00:31] falls asleep
[1518-03-16 00:44] falls asleep
[1518-04-23 00:29] wakes up
[1518-07-09 00:45] wakes up
[1518-06-30 00:44] wakes up
[1518-10-17 00:56] wakes up
[1518-05-13 00:37] falls asleep
[1518-03-08 23:58] Guard #251 begins shift
[1518-04-02 00:26] falls asleep
[1518-10-20 00:53] wakes up
[1518-03-15 00:00] Guard #3323 begins shift
[1518-09-29 00:47] falls asleep
[1518-09-21 00:41] wakes up
[1518-10-15 23:50] Guard #947 begins shift
[1518-05-01 23:59] Guard #1297 begins shift
[1518-09-17 00:17] wakes up
[1518-07-16 00:16] wakes up
[1518-11-04 00:35] wakes up
[1518-03-31 00:52] wakes up
[1518-03-03 00:43] wakes up
[1518-09-15 00:00] Guard #2113 begins shift
[1518-10-19 00:35] wakes up
[1518-08-21 00:49] falls asleep
[1518-10-27 00:38] wakes up
[1518-04-08 00:48] wakes up
[1518-07-12 23:58] Guard #251 begins shift
[1518-02-25 00:56] falls asleep
[1518-04-01 00:24] falls asleep
[1518-08-29 00:51] wakes up
[1518-04-10 00:13] wakes up
[1518-05-12 00:58] wakes up
[1518-03-01 00:10] falls asleep
[1518-06-05 00:36] wakes up
[1518-11-05 00:34] falls asleep
[1518-09-26 00:36] wakes up
[1518-06-16 00:54] falls asleep
[1518-11-22 00:50] falls asleep
[1518-02-26 23:47] Guard #1439 begins shift
[1518-03-17 00:00] Guard #3517 begins shift
[1518-07-01 00:37] wakes up
[1518-05-11 00:05] falls asleep
[1518-08-10 23:59] Guard #251 begins shift
[1518-06-13 00:30] wakes up
[1518-03-15 00:15] falls asleep
[1518-08-22 00:47] falls asleep
[1518-08-03 00:17] falls asleep
[1518-05-04 00:09] falls asleep
[1518-10-29 00:50] wakes up
[1518-02-24 00:00] Guard #1297 begins shift
[1518-09-29 00:37] falls asleep
[1518-06-11 00:02] Guard #263 begins shift
[1518-05-25 00:46] wakes up
[1518-11-05 00:36] wakes up
[1518-05-15 23:57] Guard #1033 begins shift
[1518-06-08 23:58] Guard #3469 begins shift
[1518-04-06 00:59] wakes up
[1518-06-26 00:39] falls asleep
[1518-05-23 00:03] Guard #947 begins shift
[1518-11-10 00:40] falls asleep
[1518-05-05 00:49] falls asleep
[1518-10-02 00:06] falls asleep
[1518-09-27 23:59] Guard #3517 begins shift
[1518-03-07 00:59] wakes up
[1518-05-17 00:37] falls asleep
[1518-09-30 00:32] falls asleep
[1518-07-29 00:56] wakes up
[1518-10-24 00:53] wakes up
[1518-11-22 00:21] falls asleep
[1518-03-22 00:57] falls asleep
[1518-09-29 23:59] Guard #487 begins shift
[1518-02-28 00:02] Guard #1439 begins shift
[1518-05-20 00:33] wakes up
[1518-09-13 00:57] wakes up
[1518-08-11 00:42] wakes up
[1518-07-14 00:45] wakes up
[1518-06-26 00:55] wakes up
[1518-06-01 00:09] falls asleep
[1518-07-22 00:00] Guard #3517 begins shift
[1518-06-02 23:47] Guard #2113 begins shift
[1518-09-24 23:52] Guard #2113 begins shift
[1518-11-03 00:56] wakes up
[1518-06-22 00:28] falls asleep
[1518-10-28 00:01] Guard #3209 begins shift
[1518-06-21 00:56] wakes up
[1518-06-08 00:36] wakes up
[1518-05-12 00:03] wakes up
[1518-08-09 00:54] wakes up
[1518-07-06 00:54] wakes up
[1518-05-28 00:46] falls asleep
[1518-07-30 00:45] wakes up
[1518-06-06 23:56] Guard #3517 begins shift
[1518-03-12 00:17] falls asleep
[1518-09-19 00:12] falls asleep
[1518-05-13 00:32] wakes up
[1518-05-13 00:15] falls asleep
[1518-10-17 00:35] falls asleep
[1518-08-01 00:05] falls asleep
[1518-10-31 00:00] Guard #3517 begins shift
[1518-03-31 00:42] falls asleep
[1518-05-04 00:46] falls asleep
[1518-08-22 00:00] Guard #3517 begins shift
[1518-11-06 00:12] wakes up
[1518-08-18 23:56] Guard #151 begins shift
[1518-09-02 00:36] falls asleep
[1518-05-22 00:47] wakes up
[1518-07-09 23:50] Guard #3323 begins shift
[1518-06-01 00:45] wakes up
[1518-08-08 00:42] wakes up
[1518-07-04 00:33] wakes up
[1518-08-05 00:01] Guard #1297 begins shift
[1518-03-10 23:48] Guard #1297 begins shift
[1518-10-29 00:06] falls asleep
[1518-11-05 00:47] falls asleep
[1518-05-01 00:03] Guard #907 begins shift
[1518-10-13 00:54] wakes up
[1518-09-29 00:44] wakes up
[1518-10-07 00:46] wakes up
[1518-10-10 00:21] falls asleep
[1518-08-31 00:00] Guard #1297 begins shift
[1518-03-02 00:36] wakes up
[1518-09-17 00:49] wakes up
[1518-07-16 00:46] falls asleep
[1518-06-27 00:02] Guard #907 begins shift
[1518-10-25 23:56] Guard #463 begins shift
[1518-10-08 00:52] wakes up
[1518-06-11 00:14] wakes up
[1518-11-02 00:04] falls asleep
[1518-05-06 00:45] wakes up
[1518-04-01 00:46] wakes up
[1518-09-06 00:59] wakes up
[1518-08-19 00:31] falls asleep
[1518-04-11 00:39] falls asleep
[1518-06-17 00:51] wakes up
[1518-06-08 00:54] falls asleep
[1518-09-11 00:12] wakes up
[1518-07-01 23:57] Guard #1297 begins shift
[1518-04-20 00:19] falls asleep
[1518-11-01 00:49] wakes up
[1518-11-14 00:27] wakes up
[1518-10-24 00:29] wakes up
[1518-10-20 00:20] falls asleep
[1518-05-18 00:00] falls asleep
[1518-04-06 00:01] Guard #151 begins shift
[1518-07-01 00:56] falls asleep
[1518-07-23 00:30] wakes up
[1518-06-20 00:01] Guard #1033 begins shift
[1518-07-04 23:46] Guard #251 begins shift
[1518-04-12 00:00] Guard #487 begins shift
[1518-09-29 00:00] Guard #947 begins shift
[1518-07-08 00:41] wakes up
[1518-05-14 00:53] wakes up
[1518-03-08 00:41] falls asleep
[1518-06-07 00:43] wakes up
[1518-03-13 00:39] falls asleep
[1518-04-05 00:35] wakes up
[1518-04-08 00:27] wakes up
[1518-08-10 00:00] falls asleep
[1518-05-21 00:06] wakes up
[1518-06-03 00:30] wakes up
[1518-08-06 00:48] wakes up
[1518-08-01 00:42] wakes up
[1518-06-22 00:00] Guard #3469 begins shift
[1518-08-27 00:59] wakes up
[1518-07-04 00:58] wakes up
[1518-09-05 00:20] falls asleep
[1518-07-31 00:59] wakes up
[1518-04-14 00:48] falls asleep
[1518-04-22 00:55] wakes up
[1518-05-31 00:00] Guard #907 begins shift
[1518-11-05 00:00] Guard #509 begins shift
[1518-03-09 23:54] Guard #907 begins shift
[1518-11-15 00:37] falls asleep
[1518-08-03 00:04] Guard #509 begins shift
[1518-05-26 00:33] falls asleep
[1518-09-21 00:34] falls asleep
[1518-07-30 00:04] falls asleep
[1518-05-06 00:56] falls asleep
[1518-03-25 00:18] falls asleep
[1518-09-19 00:00] Guard #1439 begins shift
[1518-08-12 23:48] Guard #1439 begins shift
[1518-03-15 00:26] wakes up
[1518-10-10 00:52] wakes up
[1518-07-14 00:02] Guard #1297 begins shift
[1518-04-13 00:51] wakes up
[1518-06-18 00:01] Guard #3469 begins shift
[1518-09-21 00:51] falls asleep
[1518-08-20 00:55] wakes up
[1518-03-19 00:05] falls asleep
[1518-10-14 00:12] wakes up
[1518-06-24 00:41] wakes up
[1518-06-12 00:52] wakes up
[1518-06-03 00:29] falls asleep
[1518-11-07 00:32] wakes up
[1518-10-08 00:34] falls asleep
[1518-03-24 00:18] falls asleep
[1518-06-02 00:29] wakes up
[1518-10-30 00:17] falls asleep
[1518-08-24 00:01] falls asleep
[1518-06-24 23:53] Guard #3323 begins shift
[1518-11-15 00:18] falls asleep
[1518-03-28 00:55] wakes up
[1518-04-30 00:57] wakes up
[1518-10-04 00:50] wakes up
[1518-08-07 00:42] falls asleep
[1518-07-27 00:50] wakes up
[1518-06-12 23:54] Guard #3469 begins shift
[1518-05-06 23:57] Guard #151 begins shift
[1518-05-04 00:32] wakes up
[1518-04-11 00:31] wakes up
[1518-04-25 00:49] wakes up
[1518-09-11 00:30] wakes up
[1518-03-04 00:38] falls asleep
[1518-10-09 00:26] falls asleep
[1518-03-02 23:58] Guard #1019 begins shift
[1518-05-28 00:25] wakes up
[1518-10-22 00:00] falls asleep
[1518-04-07 00:40] wakes up
[1518-05-17 23:52] Guard #3323 begins shift
[1518-09-07 00:00] Guard #509 begins shift
[1518-07-23 00:01] Guard #1297 begins shift
[1518-04-20 00:20] wakes up
[1518-04-22 00:03] Guard #151 begins shift
[1518-05-21 00:54] wakes up
[1518-07-30 00:44] falls asleep
[1518-09-15 00:26] falls asleep
[1518-07-04 00:44] wakes up
[1518-08-15 00:55] wakes up
[1518-11-04 00:07] wakes up
[1518-05-04 00:03] Guard #947 begins shift
[1518-09-05 23:59] Guard #463 begins shift
[1518-04-23 00:36] falls asleep
[1518-11-17 00:52] wakes up
[1518-10-24 00:27] falls asleep
[1518-11-12 00:03] falls asleep
[1518-08-22 23:47] Guard #151 begins shift
[1518-08-13 00:02] falls asleep
[1518-08-23 00:47] wakes up
[1518-04-26 23:58] Guard #1019 begins shift
[1518-05-06 00:08] falls asleep
[1518-10-31 00:07] falls asleep
[1518-05-15 00:02] Guard #1439 begins shift
[1518-07-07 00:21] falls asleep
[1518-07-10 00:02] falls asleep
[1518-05-09 00:00] falls asleep
[1518-11-08 00:59] wakes up
[1518-03-27 00:38] falls asleep
[1518-07-29 00:01] Guard #263 begins shift
[1518-06-08 00:48] falls asleep
[1518-04-09 23:49] Guard #3469 begins shift
[1518-04-24 00:54] wakes up
[1518-02-23 00:01] Guard #463 begins shift
[1518-07-19 00:03] Guard #643 begins shift
[1518-06-12 00:57] falls asleep
[1518-10-10 00:44] wakes up
[1518-07-13 00:16] falls asleep
[1518-10-13 00:48] falls asleep
[1518-04-23 00:58] wakes up
[1518-11-09 00:31] falls asleep
[1518-06-01 00:55] wakes up
[1518-06-26 00:34] wakes up
[1518-09-25 00:37] wakes up
[1518-06-04 00:00] falls asleep
[1518-10-28 00:57] falls asleep
[1518-04-19 00:54] wakes up
[1518-06-08 00:42] falls asleep
[1518-03-28 23:59] Guard #3209 begins shift
[1518-03-10 00:01] falls asleep
[1518-11-20 00:04] Guard #643 begins shift
[1518-10-29 00:24] wakes up
[1518-05-19 23:58] Guard #3209 begins shift
[1518-05-11 23:49] Guard #251 begins shift
[1518-11-01 00:00] Guard #947 begins shift
[1518-05-24 00:07] falls asleep
[1518-09-15 00:19] wakes up
[1518-09-10 00:34] wakes up
[1518-04-01 00:35] wakes up
[1518-07-08 00:27] falls asleep
[1518-05-31 00:08] falls asleep
[1518-06-15 00:04] Guard #1439 begins shift
[1518-08-17 00:00] Guard #1033 begins shift
[1518-07-12 00:00] Guard #907 begins shift
[1518-05-10 00:14] wakes up
[1518-06-08 00:04] Guard #947 begins shift
[1518-07-22 00:29] falls asleep
[1518-04-20 00:03] Guard #463 begins shift
[1518-10-21 00:47] wakes up
[1518-06-25 00:00] falls asleep
[1518-07-10 00:12] wakes up
[1518-05-31 00:47] wakes up
[1518-03-25 23:46] Guard #509 begins shift
[1518-09-18 00:46] falls asleep
[1518-07-30 00:32] wakes up
[1518-09-10 00:27] falls asleep
[1518-08-21 00:44] wakes up
[1518-09-30 00:38] wakes up
[1518-10-25 00:50] falls asleep
[1518-05-24 00:35] wakes up
[1518-03-16 00:19] falls asleep
[1518-03-16 00:54] wakes up
[1518-06-25 23:58] Guard #743 begins shift
[1518-11-13 00:54] wakes up
[1518-06-20 23:57] Guard #2113 begins shift
[1518-06-04 00:51] wakes up
[1518-08-07 00:32] wakes up
[1518-04-10 00:46] wakes up
[1518-05-14 00:47] wakes up
[1518-04-17 23:47] Guard #3323 begins shift
[1518-07-12 00:41] falls asleep
[1518-09-01 23:59] Guard #643 begins shift
[1518-05-21 00:59] wakes up
[1518-09-01 00:03] Guard #151 begins shift
[1518-05-12 00:47] falls asleep
[1518-09-23 00:03] falls asleep
[1518-09-15 00:58] wakes up
[1518-05-07 23:59] Guard #743 begins shift
[1518-04-22 00:48] falls asleep
[1518-09-18 00:01] Guard #1439 begins shift
[1518-05-16 00:39] falls asleep
[1518-06-18 00:51] wakes up
[1518-02-28 00:51] wakes up
[1518-10-02 00:10] wakes up
[1518-04-07 00:15] falls asleep
[1518-09-03 00:03] Guard #947 begins shift
[1518-04-19 00:16] falls asleep
[1518-03-26 00:25] wakes up
[1518-04-13 00:00] Guard #1307 begins shift
[1518-03-26 00:14] falls asleep
[1518-08-22 00:53] wakes up
[1518-06-18 00:27] wakes up
[1518-09-19 23:56] Guard #1033 begins shift
[1518-11-03 23:52] Guard #907 begins shift
[1518-11-07 23:57] Guard #1439 begins shift
[1518-10-04 00:41] falls asleep
[1518-09-12 00:49] falls asleep
[1518-04-24 00:34] wakes up
[1518-07-30 23:47] Guard #463 begins shift
[1518-05-10 23:53] Guard #487 begins shift
[1518-06-02 00:04] Guard #251 begins shift
[1518-05-08 00:36] wakes up
[1518-05-07 00:29] falls asleep
[1518-10-22 23:59] Guard #3469 begins shift
[1518-11-07 00:51] falls asleep
[1518-04-05 00:31] falls asleep
[1518-10-04 00:27] wakes up
[1518-11-17 23:56] Guard #463 begins shift
[1518-08-13 00:05] wakes up
[1518-03-14 00:48] wakes up
[1518-07-05 00:04] falls asleep
[1518-08-25 00:48] wakes up
[1518-04-11 00:26] falls asleep
[1518-07-17 00:03] Guard #743 begins shift
[1518-08-04 00:40] falls asleep
[1518-09-06 00:29] falls asleep
[1518-05-06 00:59] wakes up
[1518-05-17 00:53] falls asleep
[1518-05-18 00:07] wakes up
[1518-10-08 00:04] Guard #3517 begins shift
[1518-10-06 00:08] falls asleep
[1518-06-10 00:51] wakes up
[1518-10-30 00:26] wakes up
[1518-08-23 23:48] Guard #1019 begins shift
[1518-06-03 00:01] falls asleep
[1518-07-28 00:04] Guard #947 begins shift
[1518-10-28 00:52] wakes up
[1518-02-27 00:03] falls asleep
[1518-06-22 23:56] Guard #1297 begins shift
[1518-05-01 00:07] falls asleep
[1518-03-18 00:00] Guard #643 begins shift
[1518-07-20 00:09] falls asleep
[1518-03-18 23:50] Guard #463 begins shift
[1518-05-30 00:03] falls asleep
[1518-10-19 00:00] Guard #151 begins shift
[1518-09-25 00:03] falls asleep
[1518-09-08 00:38] wakes up
[1518-07-08 00:28] wakes up
[1518-08-12 00:57] wakes up
[1518-03-28 00:04] Guard #1307 begins shift
[1518-04-26 00:49] wakes up
[1518-06-15 00:40] falls asleep
[1518-08-30 00:24] falls asleep
[1518-04-01 00:53] wakes up
[1518-03-22 00:58] wakes up
[1518-08-06 00:38] falls asleep
[1518-04-06 00:26] falls asleep
[1518-09-20 00:29] falls asleep
[1518-09-12 00:27] falls asleep
[1518-10-24 00:36] falls asleep
[1518-03-24 00:51] wakes up
[1518-04-11 00:50] wakes up
[1518-04-24 00:22] falls asleep
[1518-07-04 00:49] falls asleep
[1518-09-06 00:52] falls asleep
[1518-04-24 00:51] falls asleep
[1518-06-08 00:07] falls asleep
[1518-05-18 23:57] Guard #3469 begins shift
[1518-03-15 00:49] falls asleep
[1518-05-18 00:14] falls asleep
[1518-05-28 00:48] wakes up
[1518-07-12 00:46] wakes up
[1518-08-26 00:44] wakes up
[1518-05-18 00:41] wakes up
[1518-06-28 00:04] Guard #3323 begins shift
[1518-10-05 00:03] Guard #251 begins shift
[1518-02-26 00:00] Guard #971 begins shift
[1518-09-01 00:35] falls asleep
[1518-04-18 00:50] wakes up
[1518-10-06 00:22] wakes up
[1518-08-17 23:57] Guard #643 begins shift
[1518-03-08 00:52] wakes up
[1518-11-23 00:05] falls asleep
[1518-03-20 00:03] Guard #2801 begins shift
[1518-04-17 00:38] wakes up
[1518-07-20 00:54] falls asleep
[1518-09-14 00:27] falls asleep
[1518-05-15 00:13] falls asleep
[1518-04-08 23:57] Guard #947 begins shift
[1518-08-31 00:43] falls asleep
[1518-03-13 00:59] wakes up
[1518-02-24 00:34] falls asleep
[1518-04-27 00:42] falls asleep
[1518-08-11 23:58] Guard #1307 begins shift
[1518-11-06 00:01] falls asleep
[1518-06-29 00:33] falls asleep
[1518-06-08 00:51] wakes up
[1518-03-02 00:50] falls asleep
[1518-03-11 00:51] wakes up
[1518-07-19 23:58] Guard #1307 begins shift
[1518-04-12 00:53] falls asleep
[1518-10-25 00:53] wakes up
[1518-04-29 00:50] wakes up
[1518-04-26 00:39] wakes up
[1518-06-24 00:50] falls asleep
[1518-07-26 00:01] Guard #947 begins shift
[1518-10-11 23:57] Guard #3469 begins shift
[1518-09-17 00:08] falls asleep
[1518-03-03 23:59] Guard #947 begins shift
[1518-07-27 00:10] falls asleep
[1518-09-28 00:41] wakes up
[1518-09-30 00:27] wakes up
[1518-08-06 00:13] falls asleep
[1518-06-29 00:00] Guard #1439 begins shift
[1518-10-05 00:08] falls asleep
[1518-10-14 00:40] falls asleep
[1518-06-03 00:06] wakes up
[1518-07-17 00:46] wakes up
[1518-06-18 00:18] falls asleep
[1518-09-09 00:22] falls asleep
[1518-07-08 00:38] falls asleep
[1518-08-10 00:27] wakes up
[1518-07-09 00:34] falls asleep
[1518-08-01 00:52] wakes up
[1518-10-09 00:56] wakes up
[1518-10-17 00:02] Guard #509 begins shift
[1518-07-20 00:19] wakes up
[1518-07-21 00:00] Guard #971 begins shift
[1518-03-16 00:58] wakes up
[1518-10-04 00:18] falls asleep
[1518-07-04 00:43] falls asleep
[1518-04-15 00:15] falls asleep
[1518-05-29 00:03] falls asleep
[1518-04-09 00:45] falls asleep
[1518-03-04 23:59] Guard #463 begins shift
[1518-04-18 00:38] wakes up
[1518-07-02 23:57] Guard #263 begins shift
[1518-05-17 00:42] wakes up
[1518-04-29 23:59] Guard #463 begins shift
[1518-05-13 00:44] wakes up
[1518-03-05 23:58] Guard #947 begins shift
[1518-07-07 00:02] Guard #151 begins shift
[1518-05-04 00:20] wakes up
[1518-03-14 00:29] falls asleep
[1518-08-28 23:59] Guard #263 begins shift
[1518-10-18 00:03] Guard #3041 begins shift
[1518-03-09 00:37] wakes up
[1518-07-17 23:57] Guard #1307 begins shift
[1518-09-13 00:55] falls asleep
[1518-03-20 23:57] Guard #1297 begins shift
[1518-10-17 00:21] falls asleep
[1518-06-24 00:56] wakes up
[1518-10-28 00:59] wakes up
[1518-06-19 00:31] falls asleep
[1518-05-12 00:18] falls asleep
[1518-10-23 00:28] falls asleep
[1518-07-31 23:48] Guard #509 begins shift
[1518-08-16 00:39] falls asleep
[1518-03-19 00:23] wakes up
[1518-09-08 23:58] Guard #251 begins shift
[1518-06-24 00:00] Guard #263 begins shift
[1518-11-17 00:08] falls asleep
[1518-06-13 00:45] falls asleep
[1518-10-06 23:56] Guard #151 begins shift
[1518-03-02 00:02] Guard #743 begins shift"""
from enum import Enum
import re
class Action(Enum):
GUARDID = 1
ASLEEP = 2
WAKE = 3
def inputSplit(input):
return input.split('\n')
def sortingDate(entry):
return entry[7]
def do():
splitInput = inputSplit(input)
splitInput.sort()
guards = {}
count = Action.GUARDID
guardID = 0
asleep = -1
for entry in splitInput:
entry = entry.replace('[', '')
entry = entry.replace(']', '')
values = entry.split()
date = values[0]
time = values[1]
if count == Action.GUARDID and len(values) == 4:
count = Action.ASLEEP
if count == Action.ASLEEP and len(values) > 4:
count = Action.GUARDID
if count == Action.GUARDID:
guardID = int(re.findall('[0-9]+', values[3])[0])
if guardID not in guards:
guards[guardID] = {}
count = Action.ASLEEP
continue
if count == Action.ASLEEP:
if date not in guards[guardID]:
guards[guardID][date] = [0 for x in range(60)]
asleep = int(time[3:])
count = Action.WAKE
continue
if count == Action.WAKE:
wake = int(time[3:])
for i in range(asleep, wake):
guards[guardID][date][i] = 1
count = Action.GUARDID
minutes = {}
maxTime = -1
sleepingID = -1
for guard in guards.keys():
minutes[guard] = 0
for day in guards[guard].keys():
minutes[guard] += sum(guards[guard][day])
if minutes[guard] > maxTime:
maxTime = minutes[guard]
sleepingID = guard
maxMinute = {}
maxMinuteGuardID = -1
maxMinuteSum = -1
for guard in guards.keys():
maxMinute[guard] = []
for i in range(60):
totalMinute = sum([x[i] for x in guards[guard].values()])
maxMinute[guard].append(totalMinute)
if totalMinute > maxMinuteSum:
maxMinuteSum = totalMinute
maxMinuteGuardID = guard
print(sleepingID, max(maxMinute[sleepingID]))
print(maxMinuteSum, maxMinuteGuardID)
do()<file_sep>/2022/day20.py
from collections import defaultdict
from copy import deepcopy
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
def do1(intInput):
intInput = [(seq,x) for seq,x in enumerate(intInput)]
workingList = mix(intInput, 1)
length = len(intInput)
zeroSeq = [y for (y,x) in intInput if x == 0][0]
zeroIndex = workingList.index((zeroSeq,0))
return workingList[(zeroIndex + 1000) % length][1] + workingList[(zeroIndex + 2000) % length][1] + workingList[(zeroIndex + 3000) % length][1]
def do2(intInput):
key = 811589153
intInput = [(seq, x * key) for seq,x in enumerate(intInput)]
length = len(intInput)
workingList = mix(intInput, 10)
zeroSeq = [y for (y,x) in intInput if x == 0][0]
zeroIndex = workingList.index((zeroSeq,0))
return workingList[(zeroIndex + 1000) % length][1] + workingList[(zeroIndex + 2000) % length][1] + workingList[(zeroIndex + 3000) % length][1]
def mix(intInput, times):
workingList = deepcopy(intInput)
length = len(intInput)
for _ in range(times):
for seq,number in intInput:
currentIndex = workingList.index((seq,number))
if number == 0:
continue
newIndex = (currentIndex + number) % (length - 1)
workingList.pop(currentIndex)
workingList.insert(newIndex, (seq,number))
return workingList
def do():
strInput = readInputFile(20)
intInput = convertToInt(strInput.split('\n'))
print(do1(intInput))
print(do2(intInput))
print('done')
do()<file_sep>/2018/day10.py
input = """position=< 50200, 10144> velocity=<-5, -1>
position=< -9855, -9873> velocity=< 1, 1>
position=<-29840, 30163> velocity=< 3, -3>
position=< 50213, 30162> velocity=<-5, -3>
position=< 20168, 20161> velocity=<-2, -2>
position=< 20147, 10144> velocity=<-2, -1>
position=< 30166, -39894> velocity=<-3, 4>
position=< -9863, 20157> velocity=< 1, -2>
position=< 20176, 50185> velocity=<-2, -5>
position=< 40210, -9867> velocity=<-4, 1>
position=< 40178, -29887> velocity=<-4, 3>
position=< -9840, 40171> velocity=< 1, -4>
position=<-29893, -9868> velocity=< 3, 1>
position=< 20149, -39901> velocity=<-2, 4>
position=< 30185, 40179> velocity=<-3, -4>
position=< 50171, 10147> velocity=<-5, -1>
position=< 50232, -29885> velocity=<-5, 3>
position=< 10151, 10150> velocity=<-1, -1>
position=< 30205, 30167> velocity=<-3, -3>
position=<-49871, 20158> velocity=< 5, -2>
position=< -9859, -9865> velocity=< 1, 1>
position=< 10151, -19882> velocity=<-1, 2>
position=< -9843, 10144> velocity=< 1, -1>
position=< -9825, -9865> velocity=< 1, 1>
position=< 10170, 20162> velocity=<-1, -2>
position=<-49917, 40171> velocity=< 5, -4>
position=< 40164, 40171> velocity=<-4, -4>
position=< 30198, 40171> velocity=<-3, -4>
position=< 50179, -49909> velocity=<-5, 5>
position=< 40199, -9865> velocity=<-4, 1>
position=< 10193, -39901> velocity=<-1, 4>
position=<-29880, -29886> velocity=< 3, 3>
position=< 30193, -29890> velocity=<-3, 3>
position=< -9857, 30168> velocity=< 1, -3>
position=<-29893, -29887> velocity=< 3, 3>
position=< -9843, 20159> velocity=< 1, -2>
position=< 10176, 50180> velocity=<-1, -5>
position=< 50172, -19883> velocity=<-5, 2>
position=< 40218, -29884> velocity=<-4, 3>
position=<-49911, -9870> velocity=< 5, 1>
position=<-19852, 40180> velocity=< 2, -4>
position=< -9883, -19876> velocity=< 1, 2>
position=<-29901, 30166> velocity=< 3, -3>
position=< 20160, 30171> velocity=<-2, -3>
position=< 50171, -19880> velocity=<-5, 2>
position=< 10148, -9866> velocity=<-1, 1>
position=< 20197, -49901> velocity=<-2, 5>
position=<-49895, -49902> velocity=< 5, 5>
position=< 50211, 30166> velocity=<-5, -3>
position=< 30211, 10144> velocity=<-3, -1>
position=<-19868, -39895> velocity=< 2, 4>
position=<-39867, -9870> velocity=< 4, 1>
position=<-49911, 30168> velocity=< 5, -3>
position=< 50179, 50187> velocity=<-5, -5>
position=< 50219, -29887> velocity=<-5, 3>
position=<-49895, -9874> velocity=< 5, 1>
position=< 20145, -29888> velocity=<-2, 3>
position=< 10156, 30163> velocity=<-1, -3>
position=< 20176, -49910> velocity=<-2, 5>
position=<-49863, -49902> velocity=< 5, 5>
position=<-39850, 30170> velocity=< 4, -3>
position=< 50214, -19879> velocity=<-5, 2>
position=<-19882, 30162> velocity=< 2, -3>
position=< 10160, -19878> velocity=<-1, 2>
position=< 20168, 40180> velocity=<-2, -4>
position=< 50195, 50185> velocity=<-5, -5>
position=< -9858, -9870> velocity=< 1, 1>
position=< 50187, 10153> velocity=<-5, -1>
position=<-49919, -19877> velocity=< 5, 2>
position=< 30209, 10151> velocity=<-3, -1>
position=<-49879, 20159> velocity=< 5, -2>
position=<-39902, 30171> velocity=< 4, -3>
position=< 30166, 50182> velocity=<-3, -5>
position=< -9855, 50188> velocity=< 1, -5>
position=<-39849, 50185> velocity=< 4, -5>
position=<-19884, -19880> velocity=< 2, 2>
position=<-39902, -49902> velocity=< 4, 5>
position=< 50171, -29892> velocity=<-5, 3>
position=< -9883, -39892> velocity=< 1, 4>
position=<-19850, 50184> velocity=< 2, -5>
position=<-19892, -19878> velocity=< 2, 2>
position=<-39870, -39894> velocity=< 4, 4>
position=<-39870, 40174> velocity=< 4, -4>
position=< 30196, 50189> velocity=<-3, -5>
position=<-49874, -29883> velocity=< 5, 3>
position=<-19888, 50180> velocity=< 2, -5>
position=<-49858, 10152> velocity=< 5, -1>
position=< 50192, -49905> velocity=<-5, 5>
position=<-49907, 50184> velocity=< 5, -5>
position=< 50184, 20160> velocity=<-5, -2>
position=< -9830, -19876> velocity=< 1, 2>
position=< 10159, 40172> velocity=<-1, -4>
position=< 20205, -9867> velocity=<-2, 1>
position=< 40211, -19878> velocity=<-4, 2>
position=< 20185, 10144> velocity=<-2, -1>
position=<-49875, -9865> velocity=< 5, 1>
position=< 10176, 30166> velocity=<-1, -3>
position=<-19836, 10150> velocity=< 2, -1>
position=<-19879, -29889> velocity=< 2, 3>
position=< 10183, -49905> velocity=<-1, 5>
position=< 20165, 10148> velocity=<-2, -1>
position=< 50171, -49909> velocity=<-5, 5>
position=< 30185, 20158> velocity=<-3, -2>
position=<-49915, 10148> velocity=< 5, -1>
position=<-39859, 10144> velocity=< 4, -1>
position=< 50195, -49904> velocity=<-5, 5>
position=< 40218, -39899> velocity=<-4, 4>
position=< 50183, -9874> velocity=<-5, 1>
position=< 20204, -39901> velocity=<-2, 4>
position=< 50195, -29891> velocity=<-5, 3>
position=<-39902, 20162> velocity=< 4, -2>
position=< 20152, 30162> velocity=<-2, -3>
position=<-39894, -39895> velocity=< 4, 4>
position=<-19841, -19878> velocity=< 2, 2>
position=<-19848, 30171> velocity=< 2, -3>
position=< -9870, 30168> velocity=< 1, -3>
position=< 40179, -19879> velocity=<-4, 2>
position=<-49910, -39892> velocity=< 5, 4>
position=< 10146, -9870> velocity=<-1, 1>
position=< 20197, -49902> velocity=<-2, 5>
position=<-19839, 40179> velocity=< 2, -4>
position=<-49898, -29883> velocity=< 5, 3>
position=< 20147, 20157> velocity=<-2, -2>
position=< 20176, -49905> velocity=<-2, 5>
position=< 10148, -19881> velocity=<-1, 2>
position=< 20152, 40173> velocity=<-2, -4>
position=< 20144, 20155> velocity=<-2, -2>
position=<-49858, 10151> velocity=< 5, -1>
position=< -9842, 20157> velocity=< 1, -2>
position=< 50227, 50185> velocity=<-5, -5>
position=<-29877, 50181> velocity=< 3, -5>
position=< 50203, 50189> velocity=<-5, -5>
position=< -9870, 10145> velocity=< 1, -1>
position=< -9872, 20157> velocity=< 1, -2>
position=< 20200, 40176> velocity=<-2, -4>
position=< 50227, 50182> velocity=<-5, -5>
position=< -9862, 50187> velocity=< 1, -5>
position=<-29857, 40171> velocity=< 3, -4>
position=< 30201, 40174> velocity=<-3, -4>
position=<-29900, -39897> velocity=< 3, 4>
position=<-39868, -9870> velocity=< 4, 1>
position=<-39854, -19882> velocity=< 4, 2>
position=<-29896, 10144> velocity=< 3, -1>
position=< 20168, 50183> velocity=<-2, -5>
position=< 20184, -19875> velocity=<-2, 2>
position=< -9882, -9874> velocity=< 1, 1>
position=<-29885, -39894> velocity=< 3, 4>
position=< 20184, -49901> velocity=<-2, 5>
position=<-39910, 10150> velocity=< 4, -1>
position=<-29842, -9865> velocity=< 3, 1>
position=< 10195, 40171> velocity=<-1, -4>
position=< 50187, 40174> velocity=<-5, -4>
position=< 20192, -9865> velocity=<-2, 1>
position=< -9839, -29883> velocity=< 1, 3>
position=< 10194, 20158> velocity=<-1, -2>
position=< -9827, 20160> velocity=< 1, -2>
position=<-49914, -29892> velocity=< 5, 3>
position=< 10170, 20162> velocity=<-1, -2>
position=< 10151, 40172> velocity=<-1, -4>
position=< -9846, -39892> velocity=< 1, 4>
position=< 20176, 40173> velocity=<-2, -4>
position=<-29885, 50182> velocity=< 3, -5>
position=< 10193, 30162> velocity=<-1, -3>
position=< 20160, -29884> velocity=<-2, 3>
position=<-19874, -49906> velocity=< 2, 5>
position=< -9830, 50188> velocity=< 1, -5>
position=< 20176, -9868> velocity=<-2, 1>
position=< 50211, 40176> velocity=<-5, -4>
position=<-29869, -29891> velocity=< 3, 3>
position=<-29861, 30168> velocity=< 3, -3>
position=< 50195, 50188> velocity=<-5, -5>
position=<-49903, -19882> velocity=< 5, 2>
position=<-49916, -9870> velocity=< 5, 1>
position=< 30201, -29889> velocity=<-3, 3>
position=< -9875, 50181> velocity=< 1, -5>
position=<-39910, 30170> velocity=< 4, -3>
position=< 50211, -29892> velocity=<-5, 3>
position=< 50219, 50183> velocity=<-5, -5>
position=< -9873, 40175> velocity=< 1, -4>
position=< -9855, -29891> velocity=< 1, 3>
position=<-29861, 20160> velocity=< 3, -2>
position=<-39894, 20157> velocity=< 4, -2>
position=< 30153, -29886> velocity=<-3, 3>
position=< 20171, -9867> velocity=<-2, 1>
position=< 20176, -9867> velocity=<-2, 1>
position=<-39870, -39899> velocity=< 4, 4>
position=< 30198, -29892> velocity=<-3, 3>
position=< -9854, -49901> velocity=< 1, 5>
position=< 10139, -49910> velocity=<-1, 5>
position=< -9830, -19880> velocity=< 1, 2>
position=< -9830, -39894> velocity=< 1, 4>
position=<-29880, 30162> velocity=< 3, -3>
position=< -9875, -19876> velocity=< 1, 2>
position=< 10195, -19875> velocity=<-1, 2>
position=<-29892, 40171> velocity=< 3, -4>
position=<-29893, -19883> velocity=< 3, 2>
position=< 20176, -19875> velocity=<-2, 2>
position=<-29844, -19883> velocity=< 3, 2>
position=<-29859, 10144> velocity=< 3, -1>
position=< 40211, -29887> velocity=<-4, 3>
position=<-49871, 10151> velocity=< 5, -1>
position=< 20157, -39894> velocity=<-2, 4>
position=< 30185, -9865> velocity=<-3, 1>
position=< 30196, -29892> velocity=<-3, 3>
position=< 20197, 40175> velocity=<-2, -4>
position=<-19850, 20162> velocity=< 2, -2>
position=< 30153, -9874> velocity=<-3, 1>
position=< 30153, 10148> velocity=<-3, -1>
position=<-39862, -49902> velocity=< 4, 5>
position=< 50203, -19879> velocity=<-5, 2>
position=<-39883, -39899> velocity=< 4, 4>
position=< -9862, -19880> velocity=< 1, 2>
position=< 20204, 50180> velocity=<-2, -5>
position=< 30195, -49906> velocity=<-3, 5>
position=< 10167, 10150> velocity=<-1, -1>
position=<-29865, -49901> velocity=< 3, 5>
position=< 30164, -39892> velocity=<-3, 4>
position=< -9835, -49908> velocity=< 1, 5>
position=<-19871, -19882> velocity=< 2, 2>
position=<-29853, 30168> velocity=< 3, -3>
position=<-19868, 20157> velocity=< 2, -2>
position=< 50195, -19881> velocity=<-5, 2>
position=< 40170, -49904> velocity=<-4, 5>
position=<-19851, -49910> velocity=< 2, 5>
position=< -9862, 30164> velocity=< 1, -3>
position=< 50171, -9872> velocity=<-5, 1>
position=<-39869, -19874> velocity=< 4, 2>
position=< 30180, 20155> velocity=<-3, -2>
position=< 10188, 20157> velocity=<-1, -2>
position=< 10191, -9867> velocity=<-1, 1>
position=<-29845, 10152> velocity=< 3, -1>
position=<-19889, -39901> velocity=< 2, 4>
position=< 40170, 20153> velocity=<-4, -2>
position=< 30177, 10150> velocity=<-3, -1>
position=< 30185, 20157> velocity=<-3, -2>
position=< 40206, 10144> velocity=<-4, -1>
position=<-39894, 50180> velocity=< 4, -5>
position=<-19892, 10146> velocity=< 2, -1>
position=<-39854, 10150> velocity=< 4, -1>
position=<-39889, 10151> velocity=< 4, -1>
position=< 10188, -39895> velocity=<-1, 4>
position=< 30196, 20162> velocity=<-3, -2>
position=< 10178, -29892> velocity=<-1, 3>
position=< 20184, 40178> velocity=<-2, -4>
position=< 20153, -49901> velocity=<-2, 5>
position=< 30165, 10153> velocity=<-3, -1>
position=<-49861, 30171> velocity=< 5, -3>
position=< 40186, 10149> velocity=<-4, -1>
position=<-49903, 20156> velocity=< 5, -2>
position=< 40179, 10148> velocity=<-4, -1>
position=< -9859, -19879> velocity=< 1, 2>
position=< -9827, -19880> velocity=< 1, 2>
position=<-39894, 10146> velocity=< 4, -1>
position=< 40178, 10146> velocity=<-4, -1>
position=<-19842, 10149> velocity=< 2, -1>
position=< 20192, -29884> velocity=<-2, 3>
position=<-19884, -39897> velocity=< 2, 4>
position=< 40162, 30163> velocity=<-4, -3>
position=< 50192, 40173> velocity=<-5, -4>
position=< 50173, -49906> velocity=<-5, 5>
position=< 40178, -49906> velocity=<-4, 5>
position=< 20205, -29887> velocity=<-2, 3>
position=< 30193, 10149> velocity=<-3, -1>
position=<-49871, -29886> velocity=< 5, 3>
position=<-39910, 20156> velocity=< 4, -2>
position=< -9862, -9867> velocity=< 1, 1>
position=<-19860, -49904> velocity=< 2, 5>
position=< 20176, -19883> velocity=<-2, 2>
position=< -9862, 50189> velocity=< 1, -5>
position=<-49895, 30166> velocity=< 5, -3>
position=< -9875, -29885> velocity=< 1, 3>
position=< 20184, -9873> velocity=<-2, 1>
position=<-49919, 10144> velocity=< 5, -1>
position=< -9870, 40172> velocity=< 1, -4>
position=<-49866, 30166> velocity=< 5, -3>
position=< 30165, -29888> velocity=<-3, 3>
position=<-19864, -9866> velocity=< 2, 1>
position=< 20187, 20162> velocity=<-2, -2>
position=< 20172, -29884> velocity=<-2, 3>
position=<-19876, -19879> velocity=< 2, 2>
position=< 30197, -49910> velocity=<-3, 5>
position=< 10168, -29883> velocity=<-1, 3>
position=< 10160, -19878> velocity=<-1, 2>
position=<-29893, -39892> velocity=< 3, 4>
position=<-19883, -19879> velocity=< 2, 2>
position=<-29842, 20153> velocity=< 3, -2>
position=<-39878, 30165> velocity=< 4, -3>
position=< 40223, -19874> velocity=<-4, 2>
position=<-49911, 20155> velocity=< 5, -2>
position=<-49859, -19878> velocity=< 5, 2>
position=< 50200, 10153> velocity=<-5, -1>
position=< 10145, -49910> velocity=<-1, 5>
position=< 20197, 30168> velocity=<-2, -3>
position=<-19892, -29887> velocity=< 2, 3>
position=< 10167, 30166> velocity=<-1, -3>
position=<-49903, 30168> velocity=< 5, -3>
position=< -9864, -9870> velocity=< 1, 1>
position=<-19833, 40180> velocity=< 2, -4>
position=< 50219, 50189> velocity=<-5, -5>
position=< 20197, 10153> velocity=<-2, -1>
position=<-39858, 50185> velocity=< 4, -5>
position=< 50204, -39892> velocity=<-5, 4>
position=< 40215, -49905> velocity=<-4, 5>
position=< 20168, 50180> velocity=<-2, -5>
position=<-29880, 40173> velocity=< 3, -4>
position=< -9864, 40175> velocity=< 1, -4>
position=<-39862, 10153> velocity=< 4, -1>
position=< -9854, -9865> velocity=< 1, 1>
position=<-29880, -49907> velocity=< 3, 5>
position=< -9879, -19879> velocity=< 1, 2>
position=< 50228, 10153> velocity=<-5, -1>
position=< 50187, 50183> velocity=<-5, -5>
position=< -9843, -19874> velocity=< 1, 2>
position=< 30206, 40178> velocity=<-3, -4>
position=<-29867, -49901> velocity=< 3, 5>
position=< 40165, -19879> velocity=<-4, 2>
position=< 20200, 20157> velocity=<-2, -2>
position=< -9859, 10151> velocity=< 1, -1>
position=< 20155, -39897> velocity=<-2, 4>
position=< 30154, -39901> velocity=<-3, 4>
position=< 50207, -49901> velocity=<-5, 5>
position=<-29841, -49902> velocity=< 3, 5>
position=<-39889, 20158> velocity=< 4, -2>
position=< 40173, 30162> velocity=<-4, -3>
position=< 30177, 50187> velocity=<-3, -5>
position=< 40183, 40179> velocity=<-4, -4>
position=< 40219, -29883> velocity=<-4, 3>
position=< 40182, -9870> velocity=<-4, 1>
position=< -9843, 40179> velocity=< 1, -4>
position=<-39866, 30166> velocity=< 4, -3>
position=< -9867, -49910> velocity=< 1, 5>
position=<-39877, 50189> velocity=< 4, -5>
position=<-49879, -9870> velocity=< 5, 1>
position=< 50224, -29890> velocity=<-5, 3>
position=< 40172, -49901> velocity=<-4, 5>
position=< 10151, -39893> velocity=<-1, 4>
position=< 20193, 10145> velocity=<-2, -1>
position=< 40223, -9868> velocity=<-4, 1>
position=<-49911, -19875> velocity=< 5, 2>
position=< 30174, 10144> velocity=<-3, -1>
position=< 20154, -29883> velocity=<-2, 3>
position=<-19844, 30168> velocity=< 2, -3>
position=<-19865, -49908> velocity=< 2, 5>
position=<-19843, -49909> velocity=< 2, 5>
position=< 50171, -49909> velocity=<-5, 5>
position=< 30210, 40180> velocity=<-3, -4>
position=< -9880, 50180> velocity=< 1, -5>
position=<-39870, -19875> velocity=< 4, 2>
position=< -9835, 10148> velocity=< 1, -1>
position=<-39862, -29888> velocity=< 4, 3>
position=< -9833, 30162> velocity=< 1, -3>
position=<-39852, -19874> velocity=< 4, 2>
position=<-39909, -19879> velocity=< 4, 2>
position=<-49903, 40178> velocity=< 5, -4>
position=< -9848, 50189> velocity=< 1, -5>
position=< -9831, -29891> velocity=< 1, 3>
position=<-19880, -39892> velocity=< 2, 4>
position=< 50216, -49910> velocity=<-5, 5>
position=< 50197, -39898> velocity=<-5, 4>
position=<-39897, 50185> velocity=< 4, -5>
position=< -9867, -49902> velocity=< 1, 5>
position=< 30162, -19874> velocity=<-3, 2>
position=<-19875, 40175> velocity=< 2, -4>
position=< 10156, -19878> velocity=<-1, 2>
position=< -9871, -49906> velocity=< 1, 5>
position=<-19880, 30162> velocity=< 2, -3>"""
import re
def updatePosition(coordinates):
positionX, positionY, velocityX, velocityY = coordinates
return [positionX + velocityX, positionY + velocityY, velocityX, velocityY]
def isMessage(l):
negativeCounter = 0
for star in l:
hasNeighbour = False
positionX, positionY, velocityX, velocityY = star
for neighbour in l:
positionXN, positionYN, velocityXN, velocityYN = neighbour
if (abs(positionX - positionXN) == 1 and positionY == positionYN) or (abs(positionY - positionYN) == 1 and positionX == positionXN):
hasNeighbour = True
break
if not hasNeighbour:
negativeCounter += 1
if float(negativeCounter) / len(l) < 0.2:
return True
else:
return False
def printStars(l):
minX = min([x[0] for x in l])
maxX = max([x[0] for x in l])
minY = min([x[1] for x in l])
maxY = max([x[1] for x in l])
result = [[' ' for x in range(maxX + 1)] for y in range(maxY + 1)]
for star in l:
starX = star[0] - minX
starY = star[1] - minY
result[starY][starX] = '.'
return result
def do():
splitInput = input.split('\n')
l = []
for star in splitInput:
match = re.match('.*<(.*),(.*)>.*<(.*),(.*)>', star)
positionX, positionY, velocityX, velocityY = [int(x) for x in match.groups()]
l.append([positionX, positionY, velocityX, velocityY])
seconds = 0
while(True):
seconds += 1
l = [[x[0] + x[2], x[1] + x[3], x[2], x[3]] for x in l]
if isMessage(l):
return seconds
return printStars(l)
print(do())
for line in result:
s = ''
for chr in line:
s += chr
print(s)
<file_sep>/2020/day18.py
from HelperFunctions import inputsplit
import re
def do1(puzzleInput):
sum = 0
for line in puzzleInput:
sum += calculate(line, sumUp)
return sum
def do2(puzzleInput):
sum = 0
for line in puzzleInput:
sum += calculate(line, sumWithLineBeforeDot)
return sum
def calculate(line, sumFunction):
matches = re.findall('\([0-9 \+\*]+\)', line)
while matches:
match = matches[0]
result = sumFunction(match[1:-1])
line = line.replace(match, str(result), 1)
matches = re.findall('\([0-9 \+\*]+\)', line)
return sumFunction(line)
def sumWithLineBeforeDot(line):
matches = re.findall('[\( ]?([0-9]+ \+ [0-9]+)[ \)]?', line)
while matches:
match = matches[0]
result = sumUp(match)
line = line.replace(match, str(result), 1)
matches = re.findall('[0-9]+ \+ [0-9]+', line)
return sumUp(line)
def sumUp(line):
result = 0
operation = add
for symbol in line.split(' '):
if symbol.isnumeric():
symbol = int(symbol)
result = operation(result, symbol)
elif symbol == '+':
operation = add
elif symbol == '*':
operation = multiply
return result
def multiply(result, symbol):
return result * symbol
def add(result, symbol):
return result + symbol
def do():
with open ('Input/day18.txt') as f:
strInput = f.read()
splitInput = inputsplit(strInput, '\n')
print(do1(splitInput))
print(do2(splitInput))
do()<file_sep>/2020/day11.py
from HelperFunctions import inputsplit
import copy
def do1(puzzleInput):
seatPlan = prepareInput(puzzleInput)
newSeatPlan = updateSeatPlan(seatPlan, False, 3)
return countOccupiedSeats(newSeatPlan)
def do2(puzzleInput):
seatPlan = prepareInput(puzzleInput)
newSeatPlan = updateSeatPlan(seatPlan, True, 4)
return countOccupiedSeats(newSeatPlan)
def updateSeatPlan(seatPlan, wholeLine, maxAdjacentSeats):
newSeatPlan = copy.deepcopy(seatPlan)
seatsChanged = True
while seatsChanged:
seatPlan = copy.deepcopy(newSeatPlan)
seatsChanged = False
for row in range(1, len(seatPlan) - 1):
for column in range(1, len(seatPlan[0]) - 1):
changed = newSeatState((row, column), newSeatPlan, seatPlan, wholeLine, maxAdjacentSeats)
seatsChanged = seatsChanged or changed
return newSeatPlan
def prepareInput(puzzleInput):
seatPlan = [[] for x in puzzleInput]
for i in range(len(puzzleInput)):
for j in range(len(puzzleInput[0])):
seatPlan[i].append(puzzleInput[i][j])
seatPlan[i] = ['.'] + seatPlan[i] + ['.']
floor = ['.'] + ['.' for x in puzzleInput[0]] + ['.']
seatPlan.insert(0, floor)
seatPlan.append(floor)
return seatPlan
def countOccupiedSeats(seatPlan):
count = 0
for row in seatPlan:
count += row.count('#')
return count
def newSeatState(seatPosition, newSeatPlan, seatPlan, wholeLine, maxAdjacentSeats):
row, column = seatPosition
seatState = seatPlan[row][column]
occupiedAdjacentSeats = occupiedSeats(seatPosition, seatPlan, wholeLine)
if seatState == 'L' and occupiedAdjacentSeats == 0:
newSeatPlan[row][column] = '#'
return True
elif seatState == '#' and occupiedAdjacentSeats > maxAdjacentSeats:
newSeatPlan[row][column] = 'L'
return True
return False
def occupiedSeats(seatPosition, seatPlan, wholeLine):
numberOfOccupiedSeats = 0
for stepX in [-1, 0, 1]:
for stepY in [-1, 0, 1]:
if stepX == 0 and stepY == 0:
continue
if lookForOccupiedSeats(seatPlan, seatPosition, stepX, stepY, wholeLine):
numberOfOccupiedSeats += 1
return numberOfOccupiedSeats
def lookForOccupiedSeats(seatPlan, seatPosition, stepX, stepY, wholeLine):
row, column = seatPosition
posX = row + stepX
posY = column + stepY
if wholeLine:
xEndLow = 0
yEndLow = 0
xEndHigh = len(seatPlan)
yEndHigh = len(seatPlan[0])
else:
xEndLow = row - 1
xEndHigh = row + 2
yEndLow = column - 1
yEndHigh = column + 2
while xEndLow <= posX < xEndHigh and yEndLow <= posY < yEndHigh:
if seatPlan[posX][posY] == 'L':
return False
if seatPlan[posX][posY] == '#':
return True
posX = posX + stepX
posY = posY + stepY
return False
def do():
with open ('Input/day11.txt') as f:
strInput = f.read()
splitInput = inputsplit(strInput, '\n')
print(do1(splitInput))
print(do2(splitInput))
do()<file_sep>/2021/day13.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
import re
from copy import deepcopy
def do1(dots, foldInstructions):
points,instructions = getPointsAndInstructions(dots, foldInstructions)
firstInstruction = instructions[0]
points = fold(points, [firstInstruction])
return len(points)
def do2(dots, foldInstructions):
points,instructions = getPointsAndInstructions(dots, foldInstructions)
points = fold(points, instructions)
showPoints(points)
return len(points)
def fold(points, instructions):
for instruction in instructions:
newPoints = set()
match instruction:
case ('x', value):
for x,y in points:
if x > value:
newPoints.add((value - (x - value), y))
else:
newPoints.add((x,y))
case ('y', value):
for x,y in points:
if y > value:
newPoints.add((x, value - (y - value)))
else:
newPoints.add((x,y))
points = deepcopy(newPoints)
return points
def showPoints(points):
maxX = max([x for x,y in points])
maxY = max([y for x,y in points])
grid = [['.' for i in range(maxX + 1)] for j in range(maxY + 1)]
for x,y in points:
grid[y][x] = '#'
for line in grid:
print(line)
def getPointsAndInstructions(dots, foldInstructions):
points = set()
instructions = []
for dot in dots:
x,y = convertToInt(dot.split(','))
points.add((x,y))
#foldInstructions
for line in foldInstructions:
match = re.fullmatch('fold along ([x,y])=([0-9]+)', line)
if match:
coordinate = match.groups()[0]
value = int(match.groups()[1])
instructions.append((coordinate,value))
return points,instructions
def do():
strInput = readInputFile(13)
dots,foldInstructions = strInput.split('\n\n')
dots = dots.split('\n')
foldInstructions = foldInstructions.split('\n')
print(do1(dots, foldInstructions))
print(do2(dots, foldInstructions))
print('done')
do()<file_sep>/2021/day3.py
from HelperFunctions import readInputFile
from HelperFunctions import readExampleInput
from HelperFunctions import convertToInt
from collections import defaultdict
from copy import deepcopy
import operator
def do1(splitInput):
frequency = getFrequency(splitInput)
gamma = ''
epsilon = ''
for position in sorted(frequency.keys()):
if frequency[position][0] > frequency[position][1]:
gamma += '0'
epsilon += '1'
else:
gamma += '1'
epsilon += '0'
return int(gamma, 2) * int(epsilon, 2)
def do2(splitInput):
oxygen = filterByFrequency(splitInput, operator.gt)
scrubber = filterByFrequency(splitInput, operator.le)
return scrubber * oxygen
def getFrequency(splitInput):
frequency = defaultdict(lambda: {0: 0, 1: 0})
for line in splitInput:
for position,bit in enumerate(line):
frequency[position][int(bit)] += 1
return frequency
def filterByFrequency(currentInput, compareOperator=operator.gt):
maxBit = len(currentInput[0])
for i in range(maxBit):
frequency = getFrequency(currentInput)
if compareOperator(frequency[i][0], frequency[i][1]):
currentInput = [x for x in currentInput if x[i] == '0']
else:
currentInput = [x for x in currentInput if x[i] == '1']
if len(currentInput) == 1:
break
return int(currentInput[0],2)
def do():
strInput = readInputFile(3)
splitInput = strInput.split('\n')
print(do1(splitInput))
print(do2(splitInput))
print('done')
do()<file_sep>/2018/day9.py
input = "477 players; last marble is worth 70851 points"
def do(players, marble):
result = {}
l = [0, 1]
position = 1
player = 2
for turn in range(2, marble + 1):
if (turn % 23) == 0:
if player not in result:
result[player] = 0
removePosition = (position - 7 + len(l)) % len(l)
score = turn + l[removePosition]
l.remove(l[removePosition])
result[player] += score
position = removePosition % len(l)
else:
index = (position + 2) % len(l)
l.insert(index, turn)
position = index
player = (player + 1) % players
return max(result.values())
result = do(477, 70851)
print(result) | ba3e93e4b09b283b519c3dda001ea3520a452a67 | [
"Python"
] | 94 | Python | pelma24/AdventOfCode | 6386c09620bae5f444452c62fd45a9560cfd8159 | c01d179e61102d3d8a3330ea18a1dc0b278bebd5 | |
refs/heads/master | <file_sep>/*
Navicat MySQL Data Transfer
Source Server : localhost_3306
Source Server Version : 50711
Source Host : localhost:3306
Source Database : lrfbeyond_demo
Target Server Type : MYSQL
Target Server Version : 50711
File Encoding : 65001
Date: 2016-10-31 12:20:21
*/
SET FOREIGN_KEY_CHECKS=0;
-- ----------------------------
-- Table structure for `article`
-- ----------------------------
DROP TABLE IF EXISTS `article`;
CREATE TABLE `article` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`title` varchar(100) NOT NULL,
`pubdate` int(10) NOT NULL DEFAULT '0',
PRIMARY KEY (`id`)
) ENGINE=MyISAM AUTO_INCREMENT=231 DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of article
-- ----------------------------
INSERT INTO `article` VALUES ('187', 'HTML5应用之时钟', '1346856092');
INSERT INTO `article` VALUES ('150', '自适应的网格布局-砌墙效果', '1322294653');
INSERT INTO `article` VALUES ('151', '图片延迟加载技术-Lazyload的应用', '1323418419');
INSERT INTO `article` VALUES ('152', '滚屏加载--无刷新动态加载数据技术的应用', '1324715776');
INSERT INTO `article` VALUES ('153', 'Ajax应用:使用jQuery和PHP实现功能开关效果', '1325776451');
INSERT INTO `article` VALUES ('154', 'Flowplayer-一款免费的WEB视频播放器', '1326016920');
INSERT INTO `article` VALUES ('155', 'Highcharts-功能强大的图表库的应用', '1326522351');
INSERT INTO `article` VALUES ('156', 'Highcharts选项配置详细说明文档', '1326719630');
INSERT INTO `article` VALUES ('157', '使用Highcharts生成折线图与曲线图', '1326895515');
INSERT INTO `article` VALUES ('158', '使用Highcharts生成柱状图', '1327025096');
INSERT INTO `article` VALUES ('159', '使用Highcharts结合PHP与Mysql生成饼状图', '1327918320');
INSERT INTO `article` VALUES ('160', '使用Highcharts实现柱状图、饼状图、曲线图三图合一', '1329039730');
INSERT INTO `article` VALUES ('161', 'jqGrid:强大的表格插件的应用', '1330074189');
INSERT INTO `article` VALUES ('162', 'jqGrid中文说明文档——选项设置', '1330234635');
INSERT INTO `article` VALUES ('163', 'jqGrid中文说明文档——事件和方法', '1330318428');
INSERT INTO `article` VALUES ('164', 'jqGrid表格应用——读取与查询数据', '1330591560');
INSERT INTO `article` VALUES ('165', 'jqGrid表格应用——新增与删除数据', '1330919816');
INSERT INTO `article` VALUES ('166', '使用jQuery实现跨域提交表单数据', '1331386130');
INSERT INTO `article` VALUES ('167', '浅谈响应式WEB设计', '1333072250');
INSERT INTO `article` VALUES ('168', '日期选择器:jquery datepicker的使用', '1333890332');
INSERT INTO `article` VALUES ('169', '日期时间选择器:datetimepicker', '1334306433');
INSERT INTO `article` VALUES ('170', '性能优化:如何更快速加载你的JS页面', '1335099220');
INSERT INTO `article` VALUES ('171', '使用PHP导入和导出CSV文件', '1335949304');
INSERT INTO `article` VALUES ('172', '使用PHP导入Excel和导出数据为Excel文件', '1336833793');
INSERT INTO `article` VALUES ('173', '使用PHP生成和获取XML格式数据', '1337485842');
INSERT INTO `article` VALUES ('174', 'HTML5教程和参考手册分享', '1337868595');
INSERT INTO `article` VALUES ('175', 'jQuery实现多级手风琴菜单', '1337952939');
INSERT INTO `article` VALUES ('176', '响应式WEB设计:将导航菜单转换成下拉菜单以适应小屏幕设备', '1338529515');
INSERT INTO `article` VALUES ('177', '使用HTML5的Audio标签打造WEB音频播放器', '1339598461');
INSERT INTO `article` VALUES ('178', 'Javascript+PHP实现在线拍照功能', '1340860377');
INSERT INTO `article` VALUES ('179', 'jQuery+PHP+Mysql在线拍照和在线浏览照片', '1341836937');
INSERT INTO `article` VALUES ('180', 'HTML5视频标签video', '1342165895');
INSERT INTO `article` VALUES ('181', '页面前端的水有多深?', '1342239180');
INSERT INTO `article` VALUES ('182', 'jQuery倒计时', '1342938362');
INSERT INTO `article` VALUES ('183', '使用jQuery+PHP+Mysql实现抽奖程序', '1343566149');
INSERT INTO `article` VALUES ('184', 'PHP+jQuery实现翻板抽奖', '1344063552');
INSERT INTO `article` VALUES ('185', 'HTML5之画布Canvas', '1345122224');
INSERT INTO `article` VALUES ('186', 'PHP+jQuery+Ajax+Mysql实现发表心情功能', '1345615563');
INSERT INTO `article` VALUES ('188', '基于jQuery+JSON的省市联动效果', '1347458264');
INSERT INTO `article` VALUES ('189', 'jQuery+php实现ajax文件即时上传', '1348398783');
INSERT INTO `article` VALUES ('190', '根据IP定位用户所在城市信息', '1348663533');
INSERT INTO `article` VALUES ('191', 'PHP生成各种验证码和Ajax验证', '1349525471');
INSERT INTO `article` VALUES ('192', 'HTML5应用之文件拖拽上传', '1350480671');
INSERT INTO `article` VALUES ('193', '一款基于jQuery的颜色拾取器', '1351523466');
INSERT INTO `article` VALUES ('194', 'jQuery实现页面滚动时元素智能定位', '1351688409');
INSERT INTO `article` VALUES ('195', 'jQuery+Ajax+PHP+Mysql实现分页显示数据', '1352125590');
INSERT INTO `article` VALUES ('196', 'jQuery实现返回顶部按钮效果', '1353217028');
INSERT INTO `article` VALUES ('197', 'jQuery+Ajax+PHP实现“喜欢”评级功能', '1353590248');
INSERT INTO `article` VALUES ('198', '使用jQuery和CSS将背景图片拉伸', '1353940570');
INSERT INTO `article` VALUES ('199', 'timeago.js自动将时间戳转换为更易读的时间轴', '1355158678');
INSERT INTO `article` VALUES ('200', 'HTML5+CSS3打造可自动获得焦点和支持语音输入的超酷搜索框', '1355400595');
INSERT INTO `article` VALUES ('201', 'HTML5本地存储', '1355925764');
INSERT INTO `article` VALUES ('202', '分享一款基于jQuery的QQ表情插件', '1356527449');
INSERT INTO `article` VALUES ('203', 'Javascript与HTML5的canvas实现图片旋转效果', '1357737224');
INSERT INTO `article` VALUES ('204', 'PHP递归实现无限级分类', '1358252514');
INSERT INTO `article` VALUES ('205', '使用PHPMailer发送带附件并支持HTML内容的邮件', '1359461653');
INSERT INTO `article` VALUES ('206', 'PHP+Mysql+jQuery统计当前在线用户数', '1360999500');
INSERT INTO `article` VALUES ('207', 'jQuery实现等比例缩放大图片', '1362236073');
INSERT INTO `article` VALUES ('208', '速度超快的菜单切换效果', '1363182955');
INSERT INTO `article` VALUES ('209', '使用Ctrl+Enter提交表单', '1363615171');
INSERT INTO `article` VALUES ('210', '使用PHP采集远程图片', '1363960502');
INSERT INTO `article` VALUES ('211', 'jQuery时间轴插件:jQuery Timelinr', '1364393950');
INSERT INTO `article` VALUES ('212', 'jQuery Easing 动画效果扩展', '1365428407');
INSERT INTO `article` VALUES ('213', '超酷的固定菜单页面滚动效果', '1365921498');
INSERT INTO `article` VALUES ('214', 'PHP+Mysql+jQuery实现文件下载次数统计', '1366639853');
INSERT INTO `article` VALUES ('215', '幸运大转盘-jQuery+PHP实现的抽奖程序(上)', '1367244989');
INSERT INTO `article` VALUES ('216', '幸运大转盘-jQuery+PHP实现的抽奖程序(下)', '1367501196');
INSERT INTO `article` VALUES ('217', 'jQuery数字滚动展示效果', '1368538200');
INSERT INTO `article` VALUES ('218', '砸金蛋:jQuery+PHP实现的砸金蛋中奖程序', '1369406421');
INSERT INTO `article` VALUES ('219', '响应式内容滑动插件bxSlider', '1370350238');
INSERT INTO `article` VALUES ('220', 'jQuery+HTML5声音提示', '1371218736');
INSERT INTO `article` VALUES ('221', 'WEB开发中常用的正则表达式', '1371558930');
INSERT INTO `article` VALUES ('222', 'Zclip:复制页面内容到剪贴板兼容各浏览器', '1372769186');
INSERT INTO `article` VALUES ('223', 'jQuery实现的向下推送图文信息滚动效果', '1373034355');
INSERT INTO `article` VALUES ('224', 'FileReader:读取本地图片文件并显示', '1374496739');
INSERT INTO `article` VALUES ('225', 'iPictrue:图片标注提示', '1375624096');
INSERT INTO `article` VALUES ('226', '使用jquery.qrcode生成二维码', '1375970580');
INSERT INTO `article` VALUES ('227', 'PHP汉字拼音转换和公历农历转换', '1376716887');
INSERT INTO `article` VALUES ('228', 'PHP用户注册邮箱验证激活帐号', '1377694338');
INSERT INTO `article` VALUES ('229', 'PHP+Mysql+jQuery找回密码', '1377694339');
INSERT INTO `article` VALUES ('230', '关于pagination的使用', '1323424234');
<file_sep>package com.tang.web;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.List;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.tang.service.ArticleDaoImpl;
import com.tang.vo.Article;
public class FindArticleServlet extends HttpServlet{
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
// 设置编码,规定输出内容形式
req.setCharacterEncoding("UTF-8");
resp.setContentType("text/html;charset=utf-8"); // 设置流的输出形式
resp.setCharacterEncoding("UTF-8");
PrintWriter out = resp.getWriter();
ArticleDaoImpl service = new ArticleDaoImpl();
// 设置当前页和查询数量
int pageIndex = Integer.parseInt(req.getParameter("pageIndex"));
int pageSize = Integer.parseInt(req.getParameter("pageSize"));
service.setPageIndex(pageIndex);
service.setPageSize(pageSize);
int start = service.getStart();
List<Article> list = service.findArticle(start, pageSize);
// 绘制刷新的表格
out.print("<table border='1'>");
out.print("<tr>");
out.print("<td>书号</td>");
out.print("<td>书名</td>");
out.print("<td>出版日期</td>");
for(Article article : list){
out.print("<tr>");
out.print("<td>" + article.getId() + "</td>");
out.print("<td>" + article.getTitle() + "</td>");
out.print("<td>" + article.getPubdate() + "</td>");
out.print("</tr>");
}
out.print("</table>");
//System.out.println(((List<Article>)req.getSession().getAttribute("articlelist")).size());
//resp.sendRedirect(req.getContextPath() + "/servlet/ArticleUI");
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
this.doGet(req, resp);
}
}
<file_sep># Paging
pagination实现的方式有两种,一种是全部加载数据,然后通过hidden()来实现分页,即客户端的实现</br>
还有一种是sql limit,每次查询分页的数据,即服务器端的实现。
这个例子用的是服务器分页,然后通过ajax实现无刷新的分页
<file_sep>driver=com.mysql.jdbc.Driver
url=jdbc\:mysql\://localhost\:3306/lrfbeyond_demo?useSSL\=true
username=root
password=<PASSWORD><file_sep>package com.tang.web;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.tang.service.ArticleDaoImpl;
public class ShowArticleServlet extends HttpServlet{
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
ArticleDaoImpl service = new ArticleDaoImpl();
int count = service.getArticleCount();
req.getSession().setAttribute("articlecount", count);
//req.getRequestDispatcher("../showarticle.jsp").forward(req, resp);
resp.sendRedirect("../showarticle.jsp");
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
this.doGet(req, resp);
}
}
| c8881ccb3049f7a054e8a606ea09b0a830ea8c8f | [
"Java",
"SQL",
"Markdown",
"INI"
] | 5 | SQL | TangXW777/Paging | 04d6fa42455f1951ca2a630584997852f3613eaa | a8f8258faea01a5c3bec540dfff2cfd795e558a3 | |
refs/heads/master | <repo_name>darkprogrammer/AllProjects_Code<file_sep>/KnollTextiles_source 2/TextTIlesApp/Config.h
//
// Config.h
// KnollTextiles
//
// Created by <NAME> on 1/19/14.
// Copyright (c) 2014 BMAC infotech. All rights reserved.
//
#ifndef KnollTextiles_Config_h
#define KnollTextiles_Config_h
//#define FlickrAPIKey @"ea19d7c501fe6009ad0f3e955aa16e77"
//#define FlickrSharedSecred @"56c82f9261a0f2dd"
//#define flkId @"35945744@N03"
#define FlickrAPIKey @"<KEY>"
#define FlickrSharedSecred @"1f05ff166ee06d47"
#define flkId @"55715103@N04"
#define kAuthToken @"7<PASSWORD>"
#define kRequestsAttemtsCount 3
#define kOrderMax 99999
#define kCarouselDelayTimeDuration 0.9
#define apiSig 1f05ff166ee06d47api_keyea19d7c501fe6009ad0f3e955aa16e77methodflickr.auth.getFullTokenmini_token478-358-151
#endif
<file_sep>/KnollTextiles_source 2/Constant.h
//
// Constant.h
// TextTIlesApp
//
// Created by BMAC on 07/09/13.
// Copyright (c) 2013 BMAC infotech. All rights reserved.
//
#import "HomeViewController"; | d380e6f70bc342986404284de7887df2e37206f1 | [
"C"
] | 2 | C | darkprogrammer/AllProjects_Code | 734c5fb0ab204b83f66f759c1858771a5f462f08 | 59239cd3bd9a8ce38a883ff6f7e9a902331bb1c0 | |
refs/heads/master | <repo_name>sandianshiduofen/Szwechat<file_sep>/Szwechat/line.md
login
/login/login_tel.html 注册验证电话
/login/login_addr.html 注册填写基本信息
/login/login_ok.html 注册成功
order
/order/self_help_order.html 自助下单
/order/order_succeed.html 运单提交成功
/order/send_out.html 发货记录
/order/receive.html 收货记录
/order/branch_info.html 网点详细信息
/order/share.html 运单提交成功
/order/logistics.html 发货查看物流
/order/logistics2.html 收货查看物流
/order/collection_info.html 代收款信息
ucenter
/ucenter/common_tel_edit.html 编辑常用收货电话
/ucenter/common_use_tel.html 常用收货电话
/ucenter/complain.html 网点详细信息
/ucenter/consigner_edit.html 编辑发货方
/ucenter/edit_info.html 编辑基本信息
/ucenter/qr_code.html 我的二维码
/ucenter/receiving_edit.html 编辑收货方
/ucenter/receiving_party.html 收货地址薄
/ucenter/ship_address.html 发货地址薄
/ucenter/ticket.html 运费券
/ucenter/ucenter.html 个人中心
/ucenter/select_address.html 选择常用发货地址
/ucenter/receiving_party.html 收货地址薄
<file_sep>/Szwechat/activity/common/js/store_nav.js
(function(window){
// 方法
window.incident={
// 样式
allStyle:{
navStyle:{
'height': '0.48rem',
'width':'100%',
},
liStyle:{
'display':'table-cell'
},
spanStyle:{
'width':'80px',
'margin':'0 auto',
'display':'flex',
'height':'40px',
'background': '#ffbd1f',
'text-shadow': '1px 1px 0 #ee950d',
'font-size':'0.14rem',
'color':'#fff',
'align-items': 'center',
'justify-content':'center',
'border-radius':'5px'
}
},
GetQueryString:function(name){//获取参数
var reg = new RegExp("(^|&)"+ name +"=([^&]*)(&|$)");
var r = window.location.search.substr(1).match(reg);
if(r!=null)return unescape(r[2]); return null;
},
requestTokenId:function(num){//随机数
len =num||32;
var $chars = 'ABCDEFGHJKMNPQRSTWXYZabcdefhijkmnprstwxyz2345678';
var maxPos = $chars.length;
var pwd = '';
for (i = 0; i < len; i++) {
pwd += $chars.charAt(Math.floor(Math.random() * maxPos));
}
return 'wx_tms_'+pwd;
},
userInfo:function(id){
// 创建Dom
var html='<div style="position: fixed;bottom:0;left:0;right:0;width:100%;"><ul style="display:table;width:100%"><li><span id="chitchat">聊天</span></li><li><span id="phone">拨打电话</span></li><li><span id="baguette">进店</span></li></ul></div>';
var navFooter=document.createElement('div');
navFooter.setAttribute('class', 'navFooter');
navFooter.setAttribute('id', 'navFooter');
navFooter.innerHTML=html;
// 添加样式
var navSting="";
var liSting="";
var spanSting="";
var navStyle=this.allStyle.navStyle;
var liStyle=this.allStyle.liStyle;
var spanStyle=this.allStyle.spanStyle;
for (var i in navStyle) {
navSting+=i+':'+navStyle[i]+";"
};
for (var i in liStyle) {
liSting+=i+':'+liStyle[i]+";"
};
for (var i in spanStyle) {
spanSting+=i+':'+spanStyle[i]+";"
};
navFooter.setAttribute('style', navSting);
var liDom=navFooter.children[0].children[0].children;
for (var i = 0; i < liDom.length; i++) {
liDom[i].setAttribute('style', liSting);
var spanDom=liDom[i].children[0];
spanDom.setAttribute('style', spanSting);
};
// 添加到底部
if (incident.GetQueryString('appCode')) {
document.getElementsByTagName('body')[0].appendChild(navFooter);
}
ajax({
method: 'POST',
url: 'http://weixinapi.3zqp.com/api/wx/login/findSupplier',
// url: 'http://192.168.0.158:8193/api/wx/login/findSupplier',
data: {
id:id,
garageId:incident.GetQueryString('garageId'),
requestTokenId:incident.requestTokenId()
},
success: function (response) {
var data=(typeof response == "string")?JSON.parse(response):response;
console.log(data)
if (data.returnCode==200) {
console.log(data.info)
var userinfo=JSON.stringify(data.info);
document.getElementById('chitchat').onclick=function () {
window.depot.jumpDealerFromJs(userinfo,1);
}
document.getElementById('phone').onclick=function () {
window.depot.jumpDealerFromJs(userinfo,2);
}
document.getElementById('baguette').onclick=function () {
console.log(userinfo);
window.depot.jumpDealerFromJs(userinfo,3);
}
var allPhoneBtn=document.getElementsByClassName('tel_btn');
for (var i = 0; i < allPhoneBtn.length; i++) {
allPhoneBtn[i].setAttribute('href', 'javascript:;');
allPhoneBtn[i].onclick=function(){
window.depot.jumpDealerFromJs(userinfo,2);
}
};
}else{
document.getElementById('chitchat').setAttribute('onclick', 'alert("'+data.message+'")')
document.getElementById('phone').setAttribute('onclick', 'alert("'+data.message+'")')
document.getElementById('baguette').setAttribute('onclick', 'alert("'+data.message+'")')
};
}
});
},
}
function ajax(opt) {
opt = opt || {};
opt.method = opt.method.toUpperCase() || 'POST';
opt.url = opt.url || '';
opt.async = opt.async || true;
opt.data = opt.data || null;
opt.success = opt.success || function () {};
opt.error = opt.error || function () {};
var xmlHttp = null;
if (XMLHttpRequest) {
xmlHttp = new XMLHttpRequest();
}
else {
xmlHttp = new ActiveXObject('Microsoft.XMLHTTP');
}var params = [];
for (var key in opt.data){
params.push(key + '=' + opt.data[key]);
}
var postData = params.join('&');
if (opt.method.toUpperCase() === 'POST') {
xmlHttp.open(opt.method, opt.url, opt.async);
xmlHttp.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded;charset=utf-8');
xmlHttp.send(postData);
}
else if (opt.method.toUpperCase() === 'GET') {
xmlHttp.open(opt.method, opt.url + '?' + postData, opt.async);
xmlHttp.send(null);
}
xmlHttp.onreadystatechange = function () {
if (xmlHttp.readyState == 4 && xmlHttp.status == 200) {
opt.success(xmlHttp.responseText);
}
};
}
})(window)<file_sep>/src/js/addBook.js
'use strict';
/*以下为发货地址薄*/
/*发货地址薄列表(默认)*/
function shipAddress(){
$('.header_title,.header_goback').attr('onclick', 'window.location.href="../ucenter/ucenter.html"');
loading('loading...');
$.ajax({
url: apiL+'/api/wx/address/find',
type: 'get',
dataType: 'json',
timeout : 10000,
data: {userId:userId(),type:1,requestTokenId:requestTokenId()},
success:function(data){
loadingRemove();
if (data.success) {
if (data.info.length) {
shipAddrList(data);
}else{
noshipsearch();
};
}else{
alert(data.message)
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','shipAddress()');
}else if(status=='timeout'){
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','shipAddress()');
}
}
});
}
/*没有地址列表*/
function noshipsearch(name){
if (name) {
$('.record_list').html('<div class="ship_nofind"><span><img src="../skin/images/no_find.png" alt=""></span><p>对不起,没有找到“'+name+'”,请重新输入</p></div>')
}else{
$('.record_list').html('<div class="ship_nofind"><span><img src="../skin/images/no_find.png" alt=""></span><p>对不起,您还没有添加地址</p></div>')
};
}
/*发货地址薄内容{默认} */
function shipAddrList(data){
var info = data.info;
var html="";
for(var i = 0; i < info.length; i++){
if (info[i].isDefault) {
html+='<div class="record_li ship_li" data-id="'+info[i].id+'">'
+'<table width="w100">'
+'<col width="74%">'
+'<col width="20%">'
+'<tr><td>发 货 方:'+info[i].company+'</td><td class="t_r moren"><span class="red">默认发货</span></td></tr>'
if (info[i].contact) {
html+='<tr><td colspan="2">联 系 人:'+info[i].contact+'</td></tr>'
};
html+='<tr><td colspan="2">电  话:'+info[i].phone+'<br></td></tr>'
+'<tr><td colspan="2">发货地址:'+info[i].proName+''+info[i].cityName+''+info[i].areaName+''+info[i].detailed+'<br></td></tr></table>'
+'<div class="show_details ov_h">'
+'<label class="ship_moren"></label>'
+'<a class="f_r f14" href="javascript:;" onclick="del_addr($(this),'+info[i].id+')">删除</a>'
+'<a class="f_r f14" href="../ucenter/consigner_edit.html?edit='+info[i].id+'">编辑</a>'
+'</div>'
+'</div>'
}else{
html+='<div class="record_li ship_li" data-id="'+info[i].id+'">'
+'<table width="w100" >'
+'<col width="74%">'
+'<col width="20%">'
+'<tr><td>发 货 方:'+info[i].company+'</td><td class="t_r moren"></td></tr>'
if (info[i].contact) {
html+='<tr><td colspan="2">联 系 人:'+info[i].contact+'</td></tr>'
};
html+='<tr><td colspan="2">电  话:'+info[i].phone+'<br></td></tr>'
+'<tr><td colspan="2">发货地址:'+info[i].proName+''+info[i].cityName+''+info[i].areaName+''+info[i].detailed+'<br></td></tr></table>'
+'</table>'
+'<div class="show_details ov_h">'
+'<label class="ship_moren"><input type="radio" name="default"><span class="radio"></span>设置为默认发货方</label>'
+'<a class="f_r f14" href="javascript:;" onclick="del_addr($(this),'+info[i].id+')">删除</a>'
+'<a class="f_r f14" href="../ucenter/consigner_edit.html?edit='+info[i].id+'">编辑</a>'
+'</div>'
+'</div>'
};
};
$('.record_list').html(html);
};
/*发货地址搜索(默认)*/
function receiveSearch(){
var search=$('.search_input').val();
loading('查询中...');
var data={
userId:userId(),
type:1,
searchParam:search,
requestTokenId:requestTokenId()
};
if (search){
$.ajax({
url: api+'/api/wx/address/find',
type: 'get',
dataType: 'json',
timeout : 10000,
data: data,
success:function(data){
loadingRemove();
if (data.success) {
if (data.info.length) {
shipAddrList(data);
}else{
noshipsearch(search);
};
}else{
alert(data.message)
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','receiveSearch()');
}else if(status=='timeout'){
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','receiveSearch()');
}
}
});
}else{
$.ajax({
url: apiL+'/api/wx/address/find',
type: 'get',
timeout : 10000,
dataType: 'json',
data: {userId:userId(),type:1,requestTokenId:requestTokenId()},
success:function(data){
loadingRemove();
if (data.success) {
if (data.info.length) {
shipAddrList(data);
}else{
noshipsearch();
};
}else{
alert(data.message)
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','shipAddress()');
}else if(status=='timeout'){
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','shipAddress()');
}
}
});
};
}
/*发货地址内容(自助下单)*/
function selfshipAddr(data){
var html="";
for (var i = 0; i < data.info.length; i++) {
if (data.info[i].isDefault) {
html+='<div class="record_li ship_li" data-id="'+data.info[i].id+'">'
+'<table width="w100" onclick=addrTrue('+data.info[i].id+')>'
+'<col width="74%"><col width="20%">'
+'<tr><td>发 货 方:'+data.info[i].company+'</td><td class="t_r moren"><span class="red">默认发货</span></td></tr>'
if (data.info[i].contact) {
html+='<tr><td colspan="2">联 系 人:'+data.info[i].contact+'</td></tr>'
};
html+='<tr><td colspan="2">电  话:'+data.info[i].phone+'<br></td></tr>'
+'<tr><td colspan="2">发货地址:'+data.info[i].proName+''+data.info[i].cityName+''+data.info[i].areaName+''+data.info[i].detailed+'<br></td></tr></table>'
+'<div class="show_details ov_h">'
+'<label class="ship_moren"></label>'
+'<a class="f_r f14" href="javascript:;" onclick="del_addr($(this),'+data.info[i].id+')">删除</a>'
+'<a class="f_r f14" onclick="consignerEdit('+data.info[i].id+')" href="javascript:;">编辑</a>'
+'</div>'
+'</div>'
}else{
html+='<div class="record_li ship_li" data-id="'+data.info[i].id+'">'
+'<table width="w100" onclick=addrTrue('+data.info[i].id+')>'
+'<col width="74%"><col width="20%">'
+'<tr><td>发 货 方:'+data.info[i].company+'</td><td class="t_r moren"></td></tr>'
if (data.info[i].contact) {
html+='<tr><td colspan="2">联 系 人:'+data.info[i].contact+'</td></tr>'
};
html+='<tr><td colspan="2">电  话:'+data.info[i].phone+'<br></td></tr>'
+'<tr><td colspan="2">发货地址:'+data.info[i].proName+''+data.info[i].cityName+''+data.info[i].areaName+''+data.info[i].detailed+'<br></td></tr></table>'
+'</table>'
+'<div class="show_details ov_h">'
+'<label class="ship_moren"><input type="radio" ><span class="radio"></span>设置为默认发货方</label>'
+'<a class="f_r f14" href="javascript:;" onclick="del_addr($(this),'+data.info[i].id+')">删除</a>'
+'<a class="f_r f14" onclick="consignerEdit('+data.info[i].id+')" href="javascript:;">编辑</a>'
+'</div>'
+'</div>'
};
};
$('.record_list').html(html);
}
/*发货地址薄搜索(自助下单)*/
/*function selfAddrsearch(){
var search=$('.search_input').val();
var data={
userId:userId(),
type:1,
searchParam:search,
requestTokenId:requestTokenId()
}
if (search){
$.ajax({
url: api+'/api/wx/address/find',
type: 'get',
dataType: 'json',
data: data,
success:function(data){
if (data.success) {
if (data.info.length) {
selfshipAddr(data)
}else{
noshipsearch(search);
};
}else{
alert(data.message)
};
}
});
}else{
$.ajax({
url: apiL+'/api/wx/address/find',
type: 'get',
dataType: 'json',
data: {userId:userId(),type:1,requestTokenId:requestTokenId()},
success:function(data){
if (data.success) {
if (data.info.length) {
selfshipAddr(data)
}else{
noshipsearch();
}
}else{
alert(data.message)
};
}
});
};
}*/
/*以上为发货地址薄*/
/*以下为收货地址薄*/
/*收货地址列表*/
function receivingParty(){
$('.header_title,.header_goback').attr('onclick', 'window.location.href="../ucenter/ucenter.html"');
loading('loading...');
$.ajax({
url: apiL+'/api/wx/address/find',
type: 'get',
dataType: 'json',
timeout : 10000,
data: {userId:userId(),type:2,requestTokenId:requestTokenId()},
success:function(data){
loadingRemove();
if (data.success) {
if (data.info.length) {
// console.log(data);
shipReceList(data);
}else{
noshipsearch();
};
}else{
alert(data.message)
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','receivingParty()');
}else if(status=='timeout'){
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','receivingParty()');
}
}
});
}
/*收货地址筛选搜索(默认)*/
function searchRec(){
loading('loading...');
var search=$('.search_input').val();
var data={
userId:userId(),
type:2,
searchParam:search,
requestTokenId:requestTokenId()
}
if (search){
$.ajax({
url: api+'/api/wx/address/find',
type: 'post',
timeout : 10000,
dataType: 'json',
data: data,
success:function(data){
loadingRemove();
if (data.success) {
if (data.info.length) {
shipReceList(data);
}else{
noshipsearch(search);
};
}else{
alert(data.message)
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','searchRec()');
}else if(status=='timeout'){
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','searchRec()');
}
}
});
}else{
$.ajax({
url: apiL+'/api/wx/address/find',
type: 'get',
timeout : 10000,
dataType: 'json',
data: {userId:userId(),type:2,requestTokenId:requestTokenId()},
success:function(data){
loadingRemove();
if (data.success) {
if (data.info.length) {
selfReceAddr(data)
}else{
noshipsearch();
}
}else{
alert(data.message)
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','searchRec()');
}else if(status=='timeout'){
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','searchRec()');
}
}
});
};
}
/*收货地址列表内容(默认)*/
function shipReceList(data){
var html="";
for (var i = 0; i < data.info.length; i++) {
html+='<div class="record_li ship_li" data-id="'+data.info[i].id+'">'
+'<table width="w100">'
+'<tr><td>收 货 方:'+data.info[i].company+'</td></tr>'
if (data.info[i].contact) {
html+='<tr><td colspan="2">联 系 人:'+data.info[i].contact+'</td></tr>'
};
html+='<tr><td colspan="2">电  话:'+data.info[i].phone+'<br></td></tr>'
+'<tr><td colspan="2">收货地址:'+data.info[i].proName+''+data.info[i].cityName+''+data.info[i].areaName+''+data.info[i].detailed+'<br></td></tr>'
+'</table>'
+'<div class="show_details ov_h">'
+'<a class="f_r f14" href="javascript:;" onclick="del_rec($(this),'+data.info[i].id+')">删除</a>'
+'<a class="f_r f14" href="../ucenter/receiving_edit.html?edit='+data.info[i].id+'">编辑</a>'
+'</div>'
+'</div>'
};
$('.record_list').html(html);
}
/*收货地址内容(自助下单)*/
function selfReceAddr(data){
var html="";
for (var i = 0; i < data.info.length; i++) {
html+='<div class="record_li ship_li" data-id="'+data.info[i].id+'">'
+'<table width="w100" onclick=recTrue("'+data.info[i].id+'")>'
+'<tr><td>收 货 方:'+data.info[i].company+'</td></tr>';
if (data.info[i].contact) {
html+='<tr><td colspan="2">联 系 人:'+data.info[i].contact+'</td></tr>'
};
html+='<tr><td colspan="2">电  话:'+data.info[i].phone+'<br></td></tr>'
+'<tr><td colspan="2">收货地址:'+data.info[i].proName+''+data.info[i].cityName+''+data.info[i].areaName+''+data.info[i].detailed+'<br></td></tr>'
+'</table>'
+'<div class="show_details ov_h">'
+'<a class="f_r f14" href="javascript:;" onclick="del_rec($(this),'+data.info[i].id+')">删除</a>'
+'<a class="f_r f14" onclick="recrivingEdit('+data.info[i].id+')" href="javascript:;">编辑</a>'
+'</div>'
+'</div>'
};
$('.record_list').html(html);
}
/*收货地址搜索(自助下单)*/
function selfRecsearch(){
var search=$('.search_input').val();
if (search){
var data={
userId:userId(),
type:2,
searchParam:search,
requestTokenId:requestTokenId()
};
$.ajax({
url: api+'/api/wx/address/find',
type: 'get',
dataType: 'json',
data: data,
success:function(data){
if (data.success) {
if (data.info.length) {
selfReceAddr(data);
}else{
noshipsearch(search);
};
}else{
alert(data.message)
};
}
});
}else{
$.ajax({
url: apiL+'/api/wx/address/find',
type: 'get',
dataType: 'json',
data: {userId:userId(),type:2,requestTokenId:requestTokenId()},
success:function(data){
if (data.success) {
if (data.info.length) {
selfReceAddr(data);
}else{
noshipsearch();
}
}else{
alert(data.message)
};
}
});
};
}
/*以上为收货地址薄*/
/*发货地址簿删除*/
function del_addr(e,id){
$.MsgBox.ConfirmL("确定要删除吗?",'取消','确定',function(){
$.ajax({
url: apiL+'/api/wx/address/findedit',
type: 'post',
dataType: 'json',
data: {id:id,type:1,requestTokenId:requestTokenId()},
success:function(data){
var isDefault=data.info.isDefault;
if (data.success) {
$.ajax({
url: apiL+'/api/wx/address/delete',
type: 'post',
dataType: 'json',
data: {type:1,id:id,userId:userId,isDefault:isDefault,requestTokenId:requestTokenId()},
success:function(data1){
if (data1.success) {
shipAddress();
e.parents('.record_li').remove();
}else{
alert(data1.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
fubottom('删除失败,请重试');
}else if(status=='timeout'){
fubottom('删除失败,请重试');
}
}
});
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
fubottom('删除失败,请重试');
}else if(status=='timeout'){
fubottom('删除失败,请重试');
}
}
});
},function(){
return false;
})
};
/*收地址簿删除*/
function del_rec(e,id){
$.MsgBox.ConfirmL("确定要删除吗?",'取消','确定',function(){
loading('删除中...');
$.ajax({
url: apiL+'/api/wx/address/delete',
type: 'post',
dataType: 'json',
data: {type:2,id:id,userId:userId(),isDefault:0,requestTokenId:requestTokenId()},
success:function(data){
loadingRemove();
if (data.success) {
e.parents('.record_li').remove();
if ($('.ship_li').length==0) {
$('.record_list').html('<div class="ship_nofind"><span><img src="../skin/images/no_find.png" alt=""></span><p>对不起,您还没有添加地址</p></div>')
};
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
fubottom('删除失败,请重试');
}else if(status=='timeout'){
fubottom('删除失败,请重试');
}
}
});
},function(){
return false;
})
};
function addrTrue(id){
$.ajax({
url: apiL+'/api/wx/address/findedit',
type: 'get',
dataType: 'json',
data: {type:1,id:id,requestTokenId:requestTokenId()},
success:function(data){
if (data.success) {
sessionStorage.consigner='{"company":"'+data.info.company+'","tel":'+data.info.phone+',"provs":"'+data.info.proName+'","city":"'+data.info.cityName+'","dists":"'+data.info.areaName+'","detail":"'+data.info.detailed+'","name":"'+data.info.contact+'","citynum":"'+data.info.proNumber+','+data.info.cityNumber+','+data.info.areaNumber+'"}';
if (!GetQueryString('waybill')) {
window.location.href="../order/self_help_order.html";
}else{
window.location.href="../order/self_help_order.html?waybill="+GetQueryString('selfHp')+"&amend=1";
};
}else{
alert(data.message);
};
}
});
}
function recTrue(id){
$.ajax({
url: apiL+'/api/wx/address/findedit',
type: 'get',
dataType: 'json',
data: {type:2,id:id,requestTokenId:requestTokenId()},
success:function(data){
if (data.success) {
sessionStorage.delivery='{"company":"'+data.info.company+'","tel":'+data.info.phone+',"provs":"'+data.info.proName+'","city":"'+data.info.cityName+'","dists":"'+data.info.areaName+'","detail":"'+data.info.detailed+'","name":"'+data.info.contact+'","citynum":"'+data.info.proNumber+','+data.info.cityNumber+','+data.info.areaNumber+'"}';
if (!GetQueryString('waybill')) {
window.location.href="../order/self_help_order.html";
}else{
window.location.href="../order/self_help_order.html?waybill="+GetQueryString('selfHp')+"&amend=1";
};
}else{
alert(data.message)
};
}
});
}<file_sep>/src/js/help_self.js
'use strict';
$(function() {
/*返回退出*/
/*if (!GetQueryString('waybill')) {
var ua = navigator.userAgent.toLowerCase();
if (/android/.test(ua)) {
popstate();
};
};*/
if (userId()) {
selfLoading();
}else if(!GetQueryString('code')){
document.write('<p style="text-align:center;line-height:4rem;font-size:20px;">请在微信三真驿道公众号菜单中打开</p>')
}
$('label').on('change', '.pay_way2', function(event) {
if ($(this).attr('checked')) {
/*如果绑定银行卡的操作*/
$('[name="have_coll"]').attr('onclick', 'price_open()');
price_open();
}else{
$('[name="have_coll"]').val('').removeAttr('onclick');
$('[name="goodsAmount"]').val('0');
if ($('[name="payType"]').eq(2).attr('checked')) {
$('[name="payType"]').eq(2).removeAttr('checked');
};
};
self_btn_show()
});
/*自助下单货物件数初始化*/
addno();
/*当附近的网点只有一个时,默认转中状态*/
var nearby_li=$('.nearby ul li');
if (nearby_li.length==1) {
nearby_li.find('.pay_way').attr('checked', 'checked');
};
$('table').on('click', '[name="payType"]:eq(2)', function(event) {
if ($('[name="goodsAmount"]').val()<=0) {
alert('未选择代收货款,选择回单付必须要有代收货款哦!');
return false;
}
});
/*判断是修改还是新增*/
/*付款方式变化*/
$('[name="payType"]').change(function(event) {
self_btn_show();
});
/*包裹数量变化*/
$('[name="goodsQuantity"]').change(function(event) {
self_btn_show();
});
/*货物名称变化*/
$('[name="goodsName"]').keyup(function(event) {
self_btn_show();
});
/*网点变化*/
$('.nearby').on('click', '[name="inputDotId"]', function(event) {
self_btn_show();
});
setTimeout(function(){
self_btn_show();
}, 100);
});
function selfLoading(){
if (GetQueryString("waybill")) {
$('[name="senderId"]').val(number());
$('[name="senderRegisterMobile"]').val(userName());
loading('loading...');
document.title='修改运单';
$('#selfSubmit').attr('onclick', 'auto_help_edit()');
var waybill=GetQueryString("waybill");
$.ajax({
url: apiAn+'/api/wx/waybill/getWaybill',
type: 'POST',
timeout: 10000,
dataType: 'json',
data: {waybillNo:waybill,requestTokenId:requestTokenId()},
success:function(data){
loadingRemove();
if (data.success) {
var info=data.info;
console.log(info);
// bannceEdit(info.senderCityId,info.senderAreaId,info.receiverAreaId,info.inputDotId);
$('#selfSubmit').attr('onclick', 'auto_help_edit()');
/*发货地址*/
$('[name="receiverName"]').val(info.receiverName);
$('[name="receiverLinkman"]').val(info.receiverLinkman);
$('[name="receiverMobile"]').val(info.receiverMobile);
$('[name="receiverProvinceName"]').val(info.receiverProvinceName);
$('[name="receiverCityName"]').val(info.receiverCityName);
$('[name="receiverAreaName"]').val(info.receiverAreaName);
$('[name="receiverProvinceId"]').val(info.receiverProvinceId);
$('[name="receiverCityId"]').val(info.receiverCityId);
$('[name="receiverAreaId"]').val(info.receiverAreaId);
$('[name="receiverAddress"]').val(info.receiverAddress);
$('[name="receiverId"]').val(info.receiverId);
$('[name="receiverRegisterCompany"]').val(info.receiverName);
if (info.receiverLinkman) {
var receiverLinkman=info.receiverLinkman;
}else{
var receiverLinkman='';
};
var receiverhtml='<p id="addr_receiver"> '+info.receiverName+' <br> '+receiverLinkman+' '+info.receiverMobile+' <br>'+info.receiverProvinceName+' '+info.receiverCityName+' '+info.receiverAreaName+' '+info.receiverAddress+'</p>';
$('.delivery_edit a').html(receiverhtml);
/*发货地址*/
$('[name="senderName"]').val(info.senderName);
$('[name="senderLinkman"]').val(info.senderLinkman);
$('[name="senderMobile"]').val(info.senderMobile);
$('[name="senderProvinceName"]').val(info.senderProvinceName);
$('[name="senderCityName"]').val(info.senderCityName);
$('[name="senderAreaName"]').val(info.senderAreaName);
$('[name="senderProvinceId"]').val(info.senderProvinceId);
$('[name="senderCityId"]').val(info.senderCityId);
$('[name="senderAreaId"]').val(info.senderAreaId);
$('[name="senderAddress"]').val(info.senderAddress);
if (info.senderLinkman) {
var senderLinkman=info.senderLinkman;
}else{
var senderLinkman='';
};
var senderhtml='<p id="addr_consigner"> '+info.senderName+' <br> '+senderLinkman+' '+info.senderMobile+' <br>'+info.senderProvinceName+' '+info.senderCityName+' '+info.senderAreaName+' '+info.senderAddress+'</p>';
$('.consigner_edit a').html(senderhtml);
/*商品名称*/
$('[name="goodsName"]').val(info.goodsName);
/*商品数量*/
$('[name="goodsQuantity"]').val(info.goodsQuantity);
if (info.goodsQuantity>1) {
$('#reduce').removeClass('no');
};
if (info.goodsQuantity>=999) {
$('#increase').addClass('no');
};
/*代收款金额*/
if (info.goodsAmount) {
$('[name="collection"]').attr('checked', 'checked');
var APrice=parseFloat(info.goodsAmount).toFixed(2);
var ADian=outputmoney(APrice);
$('[name="goodsAmount"]').val(info.goodsAmount);
$('[name="have_coll"]').val(ADian).attr('onclick', 'price_open()');
};
/*支付方式*/
$('[name="payType"]').eq(info.payType-1).attr('checked', 'checked');
/*判断是不是已发货*/
if (data.info.status >=1) {
bannceEdit(info.senderCityId,info.senderAreaId,info.receiverAreaId,info.inputDotId,data.info.status);
$('.consigner_edit a').removeAttr('onclick').parents('tr').css('background', '#f5f5f5');
$('.delivery_edit a').removeAttr('onclick').parents('tr').css('background', '#f5f5f5');
$('.addr_book_btn').remove();
$('[name="goodsName"]').addClass('lost').attr('readonly', 'readonly');
$('[name="goodsQuantity"]').attr('readonly', 'readonly').css({
background: '#f1f1f1',
borderColor: '#ccc',
color:'#999'
});
$('.have_coll_a').css('background', '#fff');
$('span#reduce').removeAttr('onclick').css('background-image', 'url(../skin/images/jian2.png)');
$('span#increase').removeAttr('onclick').css('background-image', 'url(../skin/images/jia2.png)');
$('[name="payType"]').attr('disabled', 'disabled');
$('#selfSubmit').attr('onclick', 'auto_help_coll()');
$('.payment:checked+span').css('border-color', '#999');
$('.payment:checked+span b').css('background', '#999');
}else{
bannceEdit(info.senderCityId,info.senderAreaId,info.receiverAreaId,info.inputDotId);
};
}else{
alert(data.message)
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
$('a').removeAttr('onclick');
alert('请求超时,请刷新重新获取');
}else if(status=='timeout'){
$('a').removeAttr('onclick');
alert('请求超时,请刷新重新获取');
}
}
});
}else{
if (userId()) {
self_load();
};
};
}
/*返回*/
function selfHeaderGoback(){
history.back(-1);
}
/*自助下单初始化包裹*/
function addno(){
var val=parseInt($('.parcel_num').val());
if (val<=1) {
$('#reduce').addClass('no');
}else if (val>=100) {
$('#increase').addClass('no');
}else{
return true;
};
}
/*自助下单加载*/
function self_load(){
/*默认一个发货方地址*/
if (userId()) {
loading('加载中...');
$.ajax({
url: api+'/api/wx/login/finduser',
type: 'POST',
dataType: 'json',
timeout : 10000,
data: {id:userId(),requestTokenId:requestTokenId()},
success:function(data){
if (data.success) {
if (!data.info.company) {
window.location.href='/login/login_addr.html?unionid='+data.info.unionid;
}else{
$.ajax({
url: apiL+'/api/wx/address/find',
type: 'post',
timeout : 10000,
dataType: 'json',
data: {type:1,userId:userId(),requestTokenId:requestTokenId()},
success:function(data){
if (data.success) {
var html="";
for (var i = 0; i < data.info.length; i++) {
if (data.info[i].isDefault==1) {
if (data.info[i].contact) {
var contact=data.info[i].contact;
}else{
var contact="";
};
html='<p id="addr_consigner"> '+data.info[i].company+' <br> '+contact+' '+data.info[i].phone+' <br>'+data.info[i].proName+' '+data.info[i].cityName+' '+data.info[i].areaName+' '+data.info[i].detailed+'</p>';
$('.consigner_edit a').html(html);
$('[name="senderAddId"]').val(data.info[i].id);
$('[name="senderAddIsDf"]').val(data.info[i].isDefault);
$('[name="senderName"]').val(data.info[i].company);
$('[name="senderLinkman"]').val(data.info[i].contact);
$('[name="senderMobile"]').val(data.info[i].phone);
$('[name="senderProvinceName"]').val(data.info[i].proName);
$('[name="senderCityName"]').val(data.info[i].cityName);
$('[name="senderAreaName"]').val(data.info[i].areaName);
$('[name="senderProvinceId"]').val(data.info[i].proNumber);
$('[name="senderCityId"]').val(data.info[i].cityNumber);
$('[name="senderAreaId"]').val(data.info[i].areaNumber);
$('[name="senderAddress"]').val(data.info[i].detailed);
};
};
}else{
alert(data.message)
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
$.MsgBox.Confirmt("默认发货地址获取失败,重新获取?",'重新获取','我自己选',function(){
return false;
},function(){
self_load();
});
}else if(status=='timeout'){
}
}
});
};
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
document.write('请求失败,请返回公众号重新进入');
}else if(status=='timeout'){
document.write('请求失败,请返回公众号重新进入');
}
}
});
$('[name="senderId"]').val(number());
$('[name="senderRegisterMobile"]').val(userName());
if ($('[name="senderName"]').val()) {
var sendCompany=$('[name="senderName"]').val();
var sendContact=$('[name="senderLinkman"]').val();
var sendPhone=$('[name="senderMobile"]').val();
var sendProName=$('[name="senderProvinceName"]').val();
var sendCityName=$('[name="senderCityName"]').val();
var sendAreaName=$('[name="senderAreaName"]').val();
var sendProNumber=$('[name="senderProvinceId"]').val();
var sendCityNumber=$('[name="senderCityId"]').val();
var sendAreaNumber=$('[name="senderAreaId"]').val();
var sendDetailed=$('[name="senderAddress"]').val();
var html='<p id="addr_consigner"> '+sendCompany+' <br> '+sendContact+' '+sendPhone+' <br>'+sendProName+' '+sendCityName+' '+sendAreaName+' '+sendDetailed+'</p>';
$('.consigner_edit a').html(html);
};
if ($('[name="receiverName"]').val()) {
var receCompany=$('[name="receiverName"]').val();
var receContact=$('[name="receiverLinkman"]').val();
var recePhone=$('[name="receiverMobile"]').val();
var receProName=$('[name="receiverProvinceName"]').val();
var receCityName=$('[name="receiverCityName"]').val();
var receAreaName=$('[name="receiverAreaName"]').val();
var receProNumber=$('[name="receiverProvinceId"]').val();
var receCityNumber=$('[name="receiverCityId"]').val();
var receAreaNumber=$('[name="receiverAreaId"]').val();
var receDetailed=$('[name="receiverAddress"]').val();
var html='<p id="addr_receiver"> '+receCompany+' <br> '+receContact+' '+recePhone+' <br>'+receProName+' '+receCityName+' '+receAreaName+' '+receDetailed+'</p>';
$('.delivery_edit a').html(html);
};
};
}
/*修改发货人*/
function consigner(){
if (!$('[name="consigner"]').val()) {
window.location.href="../ucenter/consigner_edit.html?add=selfH";
}else{
if (GetQueryString('waybill')) {
window.location.href="../ucenter/consigner_edit.html?edit=1&from=selfH&waybill=selfH&order="+GetQueryString('waybill');
}else{
window.location.href="../ucenter/consigner_edit.html?edit=1&from=selfH&order="+GetQueryString('waybill');
};
};
}
/*修改收货人*/
function delivery(){
if (!$('[name="delivery"]').val()) {
window.location.href="../ucenter/receiving_edit.html?add=selfH";
}else{
var csgId=$('[name="delivery"]').val();
if (GetQueryString('waybill')) {
window.location.href="../ucenter/receiving_edit.html?edit=1&from=selfH&waybill=selfH&order="+GetQueryString('waybill');
}else{
window.location.href="../ucenter/receiving_edit.html?edit=1&from=selfH&order="+GetQueryString('waybill');
};
};
}
/*代收价格*/
function price_open(){
if ($('[name="goodsAmount"]').val()>0) {
var haveColl=$('[name="goodsAmount"]').val();
}else{
var haveColl='';
};
var html='<div class="receive_pop_w agency_fund">'
+'<div class="receive_pop">'
+'<div class="receive_pop_c">'
+'<div class="receive_pop_close" onclick="receive_close()"></div>'
+'<div class="uc_cou_bot">'
+'<div class="receive_pop_tit">'
+'<span onclick="receive_close()"></span>'
+'</div>'
+'<div class="uc_cou_con collection_pop">'
+'<input class="price_coll" type="number" placeholder="输入代收货款金额" onblur="coll_price($(this))" oninput="if(value.length>9)value=value.slice(0,9);">'
+'<p class="msg"></p>'
+'<p>请认真核对代收款金额,运单未签收前您可自助修改金额</p>'
+'<footer>'
+'<a class="footer_btn" href="javascript:;" onclick="coll_submit()">确定</a>'
+'</footer></div></div></div></div></div>'
$('body').append(html);
var priceA=parseFloat(haveColl).toFixed(2);
/*var dianA=outputmoney(priceA);*/
$('.price_coll').val(priceA).focus();
}
function receive_close(){
$('.agency_fund').remove();
if (parseFloat($('[name="goodsAmount"]').val())<5) {
$('[name="collection"]').removeAttr('checked');
$('[name="have_coll"]').removeAttr('onclick');
};
}
/*提交收货款金额*/
function coll_submit(){
if (coll_price($('.price_coll'))) {
var val=$('.price_coll').val();
var price=parseFloat(val).toFixed(2);
var dian=outputmoney(price);
$('#have_coll').val(dian);
$('[name="goodsAmount"]').val(price);
$('.agency_fund').remove();
}else{
coll_price($('.price_coll'))
};
}
/*以下为金额转化为三个字符一个“,”逗号*/
function outputmoney(number) {
number = number.replace(/\,/g, "");
if(isNaN(number) || number == "")return "";
number = Math.round(number * 100) / 100;
if (number < 0)
return '-' + outputdollars(Math.floor(Math.abs(number) - 0) + '') + outputcents(Math.abs(number) - 0);
else
return outputdollars(Math.floor(number - 0) + '') + outputcents(number - 0);
}
/*格式化金额*/
function outputdollars(number) {
if (number.length <= 3)
return (number == '' ? '0' : number);
else {
var mod = number.length % 3;
var output = (mod == 0 ? '' : (number.substring(0, mod)));
for (var i = 0; i < Math.floor(number.length / 3); i++) {
if ((mod == 0) && (i == 0))
output += number.substring(mod + 3 * i, mod + 3 * i + 3);
else
output += ',' + number.substring(mod + 3 * i, mod + 3 * i + 3);
}
return (output);
}
}
function outputcents(amount) {
amount = Math.round(((amount) - Math.floor(amount)) * 100);
return (amount < 10 ? '.0' + amount : '.' + amount);
}
/*以上为金额转化为三个字符一个“,”逗号*/
/*验证输入有代收款是否正确*/
function coll_price(e){
var price_val=e.val();
if (price_val) {
if (!isverify(price_val)) {
$('.msg').html('<span class="red">小数点后最多保留两位小数,代收货款需低于100万</span>');
return false;
}else{
if (parseFloat(price_val)<2) {
$('.msg').html('<span class="red">代收货款金额最低为2元</span>');
return false;
}else{
$('.msg').html('');
return true;
};
};
}else{
$('.msg').html('<span class="red">请输入正确的代收货款金额</span>');
return false;
};
}
/*验证价格*/
function isverify(verify) {
var pattern = /^\d{1,6}(\.\d{1,2})?$/;
return pattern.test(verify);
}
/*货物件数变化时限制*/
function num_change(e){
if (e.val()<=1) {
e.val('1');
$('#reduce').addClass('no');
$('#increase').removeClass('no');
return false;
}else if(e.val()>=999){
e.val('999');
$('#reduce').removeClass('no');
$('#increase').addClass('no');
return false;
}else{
$('#reduce').removeClass('no');
$('#increase').removeClass('no');
}
if(!/^\d+$/.test(e.val())){
var intval=parseInt(e.val())
if (!isNaN(intval)) {
e.val(intval);
if (intval==1) {
$('#reduce').addClass('no');
$('#increase').removeClass('no');
}
}else{
e.val(1);
$('#reduce').addClass('no');
$('#increase').removeClass('no');
};
}
self_btn_show();
}
/*减少包裹*/
function reduce(e){
var val=parseInt(e.siblings('.parcel_num').val());
if (val<=1) {
e.addClass('no');
}else{
val--;
if (val==1) {
e.addClass('no');
};
$('#increase').removeClass('no');
};
e.siblings('.parcel_num').val(val);
}
/*增加包裹*/
function increase(e){
var val=parseInt(e.siblings('.parcel_num').val());
if (val>=999) {
e.addClass('no');
}else{
val++;
if (val==999) {
e.addClass('no');
};
$('#reduce').removeClass('no');
};
e.siblings('.parcel_num').val(val);
}
/*提交运单*/
function self_submit(){
var consigner=$('input[name="senderName"]').val();
var delivery=$('input[name="receiverName"]').val();
var goodsName=$('input[name="goodsName"]').val();
var branch=$('input[name="inputDotId"]:checked').val();
var pay_way=$('input[name="payType"]:checked').val();
var have_coll="1";
if ($('.pay_way2').attr('checked')) {
have_coll=$('.have_coll').val();
}
if (!consigner) {
$.MsgBox.Alert("请填写发货人的联系方式和详细信息",'确定');
}else if(!delivery){
$.MsgBox.Alert("请填写收货人的联系方式和详细信息",'确定');
}else if(!goodsName){
$.MsgBox.Alert("请填写货物信息",'确定');
}/*else if(!pay_way){
$.MsgBox.Alert("请选择付款方式",'确定');
}*/else if(!have_coll){
$.MsgBox.Alert("请输入代收货款金额",'确定');
}else if(!branch){
$.MsgBox.Alert("请选择发货网点",'确定');
}else{
protocol();
};
}
function self_btn_show(){
var consigner=$('input[name="senderName"]').val();
var delivery=$('input[name="receiverName"]').val();
var goodsName=trim($('input[name="goodsName"]').val());
var branch=$('input[name="inputDotId"]:checked').val();
// var pay_way=$('input[name="payType"]:checked').val();
var goodsQuantity=$('input[name="goodsQuantity"]').val();
if (consigner&&delivery&&goodsName&&branch&&goodsQuantity/*&&pay_way*/) {
$('.footer_self .footer_btn').removeClass('hui').removeAttr('disabled');
}else{
$('.footer_self .footer_btn').addClass('hui').attr('disabled', 'disabled');
};
}
/*提交后弹出协议*/
function protocol(){
$('.protocol_w').css('display', 'block');
}
/*提交关闭协议*/
function protocol_close(){
$('.protocol_w').css('display', 'none');
}
/*协议确认提交*/
function auto_help_ok(){
loading('提交中...');
$.ajax({
url: apiAn+'/api/wx/waybill/insertWaybill',
type: 'POST',
timeout : 10000,
dataType: 'json',
data: $('#selfHelpOrder').serialize(),
success:function(data){
loadingRemove();
if (data.success) {
$('#selfSubmit').attr('disabled', 'disabled');
var timer=setTimeout(function(){
clearTimeout(timer);
$('#selfSubmit').removeAttr('disabled');
}, 5000);
var order=data.info;
window.location.href='../order/order_succeed.html?order='+order;
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
$('[name="requestTokenId"]').val(requestTokenId());
loadingRemove();
if(status=='error'){
alert('提交失败,请重试');
}else if(status=='timeout'){
alert('提交失败,请重试');
}
}
});
}
/*自助下单修改*/
function auto_help_edit(){
loading('提交中...');
var waybillNo=GetQueryString('waybill');
$.ajax({
url: apiAn+'/api/wx/waybill/editWaybill',
type: 'POST',
timeout : 10000,
dataType: 'json',
data: $('#selfHelpOrder').serialize()+'&no='+waybillNo,
success:function(data){
loadingRemove();
if (data.success) {
$('#selfSubmit').attr('disabled', 'disabled').css('background', '#999');
var timer=setTimeout(function(){
clearTimeout(timer);
$('#selfSubmit').removeAttr('disabled').css('background', '#52BB03');
}, 5000);
var order=data.info;
window.location.href='../order/scan.html?order='+order;
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
$('[name="requestTokenId"]').val(requestTokenId());
loadingRemove();
if(status=='error'){
fubottom('修改失败,请重试');
}else if(status=='timeout'){
fubottom('修改失败,请重试');
}
}
});
}
/*自助下单修改*/
function auto_help_coll(){
loading('提交中...');
var goodsAmount=$('[name="goodsAmount"]').val();
var waybillNo=GetQueryString('waybill');
var data={
no:waybillNo,
waybillNo:waybillNo,
goodsAmount:goodsAmount,
requestTokenId:requestTokenId()
};
$.ajax({
url: apiAn+'/api/wx/waybill/editWaybillGoodsAmount',
type: 'POST',
timeout : 10000,
dataType: 'json',
data: data,
success:function(data){
loadingRemove();
if (data.success) {
$('#selfSubmit').attr('disabled', 'disabled').css('background', '#999');
var timer=setTimeout(function(){
clearTimeout(timer);
$('#selfSubmit').removeAttr('disabled').css('background', '#52BB03');
}, 5000);
var order=data.info;
window.location.href='../order/scan.html?order='+order;
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
fubottom('修改失败,请重试');
}else if(status=='timeout'){
fubottom('修改失败,请重试');
}
}
});
}
/*附近网点列表*/
function bannce(cityId,areaId,receiverAreaId){
if (areaId) {
$.ajax({
url: apiAn+'/api/wx/dot/getListByArea',
type: 'POST',
timeout : 10000,
dataType: 'json',
data: {cityId:cityId,areaId:areaId,receiverAreaId:receiverAreaId,requestTokenId:requestTokenId()},
success:function(data){
if (data.success) {
// console.log(data);
if (data.info.length>0) {
var html='<h6>附近可揽货网点:共为您匹配到<span class="red">'+data.info.length+'</span>家可揽货网点</h6>';
html+='<ul>';
var nowData=new Date();
for (var i = 0; i < data.info.length; i++) {
var startBusinessHour=(data.info[i].startBusinessHour).slice(0,5);
var endBusinessHour=(data.info[i].endBusinessHour).slice(0,5);
if (data.info[i].inBusiness) {
html+='<li data-id="'+data.info[i].id+'">';
}else{
html+='<li class="branch_close" data-id="'+data.info[i].id+'">';
};
html+='<label><div class="branch_w">'
+'<div class="branch_l">'
+'<p><input class="pay_way branch" id="branch'+data.info[i].id+'" type="radio" name="inputDotId" value="'+data.info[i].id+'"><span><b></b></span>'+ data.info[i].name+'</p>'
+'<p> </p>'
+'</div>'
+'<div class="branch_r">'
+'<span>揽货时间:<br>'+ startBusinessHour+'--'+ endBusinessHour+'</span>'
+'</div>'
+'</div>'
+'<p>营业地址:'+ data.info[i].province+''+ data.info[i].city+''+ data.info[i].area+''+ data.info[i].address+'</p>'
+'</label><a href="tel:'+data.info[i].tel1+'" class="blue DotTel">'+ data.info[i].tel1+'</a>'
+'</li>';
};
html+='</ul>';
$('.nearby').html(html);
}else{
var html='<h6>附近可揽货网点:共为您匹配到<span class="red">'+data.info.length+'</span>家可揽货网点</h6>';
$('.nearby').html(html);
$.MsgBox.Alert(data.message,'确定');
};
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
noResultErr($('.nearby'),200,'获取网点失败,请点击重新获取','bannce('+cityId+','+areaId+','+receiverAreaId+')');
}else if(status=='timeout'){
noResultErr($('.nearby'),200,'获取网点失败,请点击重新获取','bannce('+cityId+','+areaId+','+receiverAreaId+')');
}
}
});
return true;
};
}
/*附近网点列表*/
function bannceEdit(cityId,areaId,receiverAreaId,id,status){
var statu=status;
if (areaId) {
$.ajax({
url: apiAn+'/api/wx/dot/getListByArea',
type: 'POST',
timeout : 10000,
dataType: 'json',
data: {cityId:cityId,areaId:areaId,receiverAreaId:receiverAreaId,requestTokenId:requestTokenId()},
success:function(data){
if (data.success) {
if (!status) {
var html='<h6>附近可揽货网点:共为您匹配到<span class="red">'+data.info.length+'</span>家可揽货网点</h6>';
html+='<ul>';
var nowData=new Date();
for (var i = 0; i < data.info.length; i++) {
var startBusinessHour=(data.info[i].startBusinessHour).slice(0,5);
var endBusinessHour=(data.info[i].endBusinessHour).slice(0,5);
if (data.info[i].inBusiness) {
html+='<li data-id="'+data.info[i].id+'">';
}else{
html+='<li class="branch_close" data-id="'+data.info[i].id+'">';
};
html+='<label><div class="branch_w">'
+'<div class="branch_l">';
html+='<p><input class="pay_way branch" id="branch'+data.info[i].id+'" type="radio" name="inputDotId" value="'+data.info[i].id+'"><span><b></b></span>'+ data.info[i].name+'</p>';
html+='<p> </p>'
+'</div>'
+'<div class="branch_r">'
+'<span>揽货时间:<br>'+ startBusinessHour+'--'+ endBusinessHour+'</span>'
+'</div>'
+'</div>'
+'<p>营业地址:'+ data.info[i].province+''+ data.info[i].city+''+ data.info[i].area+''+ data.info[i].address+'</p>'
+'</label><a href="tel:'+data.info[i].tel1+'" class="blue DotTel">'+ data.info[i].tel1+'</a>'
+'</li>';
};
}else{
var html='<h6>附近可揽货网点:共为您匹配到<span class="red">1</span>家可揽货网点</h6>';
html+='<ul>';
var nowData=new Date();
$.ajax({
url: apiAn+'/api/wx/dot/getDotById',
type: 'post',
timeout : 10000,
dataType: 'json',
async:false,
data: {dotId:id,requestTokenId:requestTokenId()},
success:function(data){
// console.log(data);
if (data.success) {
var info=data.info;
var startBusinessHour=(info.startBusinessHour).slice(0,5);
var endBusinessHour=(info.endBusinessHour).slice(0,5);
if (info.inBusiness) {
html+='<li data-id="'+info.id+'">';
}else{
html+='<li class="branch_close" data-id="'+info.id+'">';
};
html+='<label><div class="branch_w">'
+'<div class="branch_l">';
html+='<p><input class="pay_way branch" id="branch'+info.id+'" type="radio" name="inputDotId" value="'+info.id+'" disabled="disabled"><span><b></b></span>'+ info.name+'</p>';
html+='<p> </p>'
+'</div>'
+'<div class="branch_r">'
+'<span>揽货时间:<br>'+ startBusinessHour+'--'+ endBusinessHour+'</span>'
+'</div>'
+'</div>'
+'<p>营业地址:'+ info.province+''+ info.city+''+ info.area+''+ info.address+'</p>'
+'</label><a href="tel:'+data.info[i].tel1+'" class="blue DotTel">'+ data.info[i].tel1+'</a>'
+'</li>';
}else{
alert(data.message)
}
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
noResultErr($('.nearby'),200,'获取网点失败,请点击重新获取','bannceEdit('+cityId+','+areaId+','+receiverAreaId+','+id+','+statu+')');
}else if(status=='timeout'){
noResultErr($('.nearby'),200,'获取网点失败,请点击重新获取','bannceEdit('+cityId+','+areaId+','+receiverAreaId+','+id+','+statu+')');
}
}
});
};
html+='</ul>';
$('.nearby').html(html);
$('#branch'+id).attr('checked', 'checked');
if (status) {
$('.branch:checked+span').css('border-color', '#999');
$('.branch:checked+span b').css('background', '#999');
};
self_btn_show();
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
noResultErr($('.nearby'),200,'获取网点失败,请点击重新获取','bannceEdit('+cityId+','+areaId+','+receiverAreaId+','+id+','+statu+')');
}else if(status=='timeout'){
noResultErr($('.nearby'),200,'获取网点失败,请点击重新获取','bannceEdit('+cityId+','+areaId+','+receiverAreaId+','+id+','+statu+')');
}
}
});
};
}
/*附近网点列表*/
function bannceEdit(cityId,areaId,receiverAreaId,id,status){
var statu=status;
if (areaId) {
if (!status) {
$.ajax({
url: apiAn+'/api/wx/dot/getListByArea',
type: 'POST',
timeout : 10000,
dataType: 'json',
data: {cityId:cityId,areaId:areaId,receiverAreaId:receiverAreaId,requestTokenId:requestTokenId()},
success:function(data){
if (data.success) {
var html='<h6>附近可揽货网点:共为您匹配到<span class="red">'+data.info.length+'</span>家可揽货网点</h6>';
html+='<ul>';
var nowData=new Date();
for (var i = 0; i < data.info.length; i++) {
var startBusinessHour=(data.info[i].startBusinessHour).slice(0,5);
var endBusinessHour=(data.info[i].endBusinessHour).slice(0,5);
if (data.info[i].inBusiness) {
html+='<li data-id="'+data.info[i].id+'">';
}else{
html+='<li class="branch_close" data-id="'+data.info[i].id+'">';
};
html+='<label><div class="branch_w">'
+'<div class="branch_l">';
html+='<p><input class="pay_way branch" id="branch'+data.info[i].id+'" type="radio" name="inputDotId" value="'+data.info[i].id+'"><span><b></b></span>'+ data.info[i].name+'</p>';
html+='<p> </p>'
+'</div>'
+'<div class="branch_r">'
+'<span>揽货时间:<br>'+ startBusinessHour+'--'+ endBusinessHour+'</span>'
+'</div>'
+'</div>'
+'<p>营业地址:'+ data.info[i].province+''+ data.info[i].city+''+ data.info[i].area+''+ data.info[i].address+'</p>'
+'</label><a href="tel:'+data.info[i].tel1+'" class="blue DotTel">'+ data.info[i].tel1+'</a>'
+'</li>';
};
html+='</ul>';
$('.nearby').html(html);
$('#branch'+id).attr('checked', 'checked');
if (status) {
$('.branch:checked+span').css('border-color', '#999');
$('.branch:checked+span b').css('background', '#999');
};
self_btn_show();
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
noResultErr($('.nearby'),200,'获取网点失败,请点击重新获取','bannceEdit('+cityId+','+areaId+','+receiverAreaId+','+id+','+statu+')');
}else if(status=='timeout'){
noResultErr($('.nearby'),200,'获取网点失败,请点击重新获取','bannceEdit('+cityId+','+areaId+','+receiverAreaId+','+id+','+statu+')');
}
}
});
}else{
$.ajax({
url: apiAn+'/api/wx/dot/getDotById',
type: 'post',
timeout : 10000,
dataType: 'json',
async:false,
data: {dotId:id,requestTokenId:requestTokenId()},
success:function(data){
// console.log(data);
if (data.success) {
var html='<h6>附近可揽货网点:共为您匹配到<span class="red">1</span>家可揽货网点</h6>';
html+='<ul>';
var nowData=new Date();
var info=data.info;
var startBusinessHour=(info.startBusinessHour).slice(0,5);
var endBusinessHour=(info.endBusinessHour).slice(0,5);
if (info.inBusiness) {
html+='<li data-id="'+info.id+'">';
}else{
html+='<li class="branch_close" data-id="'+info.id+'">';
};
html+='<label><div class="branch_w">'
+'<div class="branch_l">';
html+='<p><input class="pay_way branch" id="branch'+info.id+'" type="radio" name="inputDotId" value="'+info.id+'" disabled="disabled"><span><b></b></span>'+ info.name+'</p>';
html+='<p><a href="tel:'+info.tel1+'" class="blue">'+ info.tel1+'</a></p>'
+'</div>'
+'<div class="branch_r">'
+'<span>揽货时间:<br>'+ startBusinessHour+'--'+ endBusinessHour+'</span>'
+'</div>'
+'</div>'
+'<p>营业地址:'+ info.province+''+ info.city+''+ info.area+''+ info.address+'</p>'
+'</label>'
+'</li>';
html+='</ul>';
$('.nearby').html(html);
$('#branch'+id).attr('checked', 'checked');
if (status) {
$('.branch:checked+span').css('border-color', '#999');
$('.branch:checked+span b').css('background', '#999');
};
self_btn_show();
}else{
alert(data.message)
}
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
noResultErr($('.nearby'),200,'获取网点失败,请点击重新获取','bannceEdit('+cityId+','+areaId+','+receiverAreaId+','+id+','+statu+')');
}else if(status=='timeout'){
noResultErr($('.nearby'),200,'获取网点失败,请点击重新获取','bannceEdit('+cityId+','+areaId+','+receiverAreaId+','+id+','+statu+')');
}
}
});
};
};
}
/*发货地址薄*/
function consignerBook(){
if (!GetQueryString('waybill')) {
window.location.href="../ucenter/ship_address.html?selfHp="+GetQueryString('waybill');
}else{
window.location.href="../ucenter/ship_address.html?selfHp="+GetQueryString('waybill')+"&waybill=1";
};
}
/*收货地址薄*/
function deliveryBook(){
if (!GetQueryString('waybill')) {
window.location.href="../ucenter/receiving_party.html?selfHp="+GetQueryString('waybill');
}else{
window.location.href="../ucenter/receiving_party.html?selfHp="+GetQueryString('waybill')+"&waybill=addr";
};
}
/*移除浮层*/
function selfFaEdit(e){
e.parents('.self_help_w').remove();
}
/*移除浮层*/
function selfShouEdit(e){
e.parents('.self_help_w').remove();
}
/*发货地址提交*/
function selfShipSubmit(){
if (!noNullfa($('[name="client"]'),'单位名称')) {
noNullfa($('[name="client"]'),'单位名称');
return false;
}else if(!telNo($('[name="tel"]'))){
telNo($('[name="tel"]'))
return false;
}else if(!noNull($('[name="aeraNum"]'),'所在省、市、区')){
noNull($('[name="aeraNum"]'),'所在省、市、区');
return false;
}else if(!noNullfa($('[name="detail"]'),'详细地址')){
noNullfa($('[name="detail"]'),'详细地址');
return false;
}else{
loading('提交中...');
var senderAddId=$('[name="senderAddId"]').val();
var senderAddIsDf=$('[name="senderAddIsDf"]').val();
var company=trim($('[name="client"]').val());
var contact=trim($('[name="name"]').val());
var phone=trim($('[name="tel"]').val());
var aera=$('#addr_aera').html();
var aeraNum=$('[name="aeraNum"]').val();
var detailed=trim($('[name="detail"]').val());
var aeraArr=aera.split(' ');
var aeraNumArr=aeraNum.split(',');
if (senderAddIsDf==1) {
var isDefault=1;
}else{
var isDefault=0;
};
// console.log(isDefault);
if (Recdistinctive()) {
if (!senderAddId) {
AMap.service('AMap.Geocoder',function(){
var geocoder = new AMap.Geocoder({});
geocoder.getLocation(aeraArr[0]+""+aeraArr[1]+""+aeraArr[2]+""+detailed, function(status, result) {
loadingRemove();
if (status === 'complete' && result.info === 'OK') {
loading('提交中...');
var data={
userId:userId(),
type:1,
company:company,
contact:contact,
phone:phone,
proName:aeraArr[0],
proNumber:aeraNumArr[0],
cityName:aeraArr[1],
cityNumber:aeraNumArr[1],
areaName:aeraArr[2],
areaNumber:aeraNumArr[2],
detailed:detailed,
lat:result.geocodes[0].location.lat,
lng:result.geocodes[0].location.lng,
isDefault:isDefault,
requestTokenId:requestTokenId()
};
$.ajax({
url: apiL+'/api/wx/address/add',
type: 'get',
timeout : 10000,
dataType: 'json',
data: data,
success:function(data){
loadingRemove();
if (data.success) {
// console.log(data)
$('[name="senderAddId"]').val(data.info.id);
$('[name="senderAddIsDf"]').val(data.info.isDefault);
$('[name="senderName"]').val(company);
$('[name="senderLinkman"]').val(contact);
$('[name="senderMobile"]').val(phone);
$('[name="senderProvinceName"]').val(aeraArr[0]);
$('[name="senderCityName"]').val(aeraArr[1]);
$('[name="senderAreaName"]').val(aeraArr[2]);
$('[name="senderProvinceId"]').val(aeraNumArr[0]);
$('[name="senderCityId"]').val(aeraNumArr[1]);
$('[name="senderAreaId"]').val(aeraNumArr[2]);
$('[name="senderAddress"]').val(detailed);
var html='<p id="addr_consigner"> '+company+' <br> '+contact+' '+phone+' <br>'+aeraArr[0]+' '+aeraArr[1]+' '+aeraArr[2]+' '+detailed+'</p>';
$('.consigner_edit a').html(html);
$('.self_fa_edit').remove();
var receiverAreaId=$('[name="receiverAreaId"]').val();
if (receiverAreaId) {
if (bannce(aeraNumArr[1],aeraNumArr[2],receiverAreaId)) {
setTimeout(function(){
self_btn_show();
}, 100);
};
};
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
$('.selfShipSubmit').removeAttr('disabled');
fubottom('提交失败,请重试');
}else if(status=='timeout'){
$('.selfShipSubmit').removeAttr('disabled');
fubottom('提交失败,请重试');
}
}
});
/*防止多次提交*/
$('.selfShipSubmit').attr('disabled', 'disabled');
setTimeout(function(){
$('.selfShipSubmit').removeAttr('disabled');
}, 5000);
}else{
AMap.service('AMap.Geocoder',function(){//回调函数
//实例化Geocoder
var geocoder = new AMap.Geocoder({
// city: "010"//城市,默认:“全国”
});
geocoder.getLocation(aeraArr[0]+""+aeraArr[1]+""+aeraArr[2], function(status, result) {
loadingRemove();
if (status === 'complete' && result.info === 'OK') {
loading('提交中...');
var data={
userId:userId(),
type:1,
company:company,
contact:contact,
phone:phone,
proName:aeraArr[0],
proNumber:aeraNumArr[0],
cityName:aeraArr[1],
cityNumber:aeraNumArr[1],
areaName:aeraArr[2],
areaNumber:aeraNumArr[2],
detailed:detailed,
lat:result.geocodes[0].location.lat,
lng:result.geocodes[0].location.lng,
isDefault:isDefault,
requestTokenId:requestTokenId()
};
$.ajax({
url: apiL+'/api/wx/address/add',
type: 'get',
timeout : 10000,
dataType: 'json',
data: data,
success:function(data){
loadingRemove();
if (data.success) {
// console.log(data)
$('[name="senderAddId"]').val(data.info.id);
$('[name="senderAddIsDf"]').val(data.info.isDefault);
$('[name="senderName"]').val(company);
$('[name="senderLinkman"]').val(contact);
$('[name="senderMobile"]').val(phone);
$('[name="senderProvinceName"]').val(aeraArr[0]);
$('[name="senderCityName"]').val(aeraArr[1]);
$('[name="senderAreaName"]').val(aeraArr[2]);
$('[name="senderProvinceId"]').val(aeraNumArr[0]);
$('[name="senderCityId"]').val(aeraNumArr[1]);
$('[name="senderAreaId"]').val(aeraNumArr[2]);
$('[name="senderAddress"]').val(detailed);
var html='<p id="addr_consigner"> '+company+' <br> '+contact+' '+phone+' <br>'+aeraArr[0]+' '+aeraArr[1]+' '+aeraArr[2]+' '+detailed+'</p>';
$('.consigner_edit a').html(html);
$('.self_fa_edit').remove();
var receiverAreaId=$('[name="receiverAreaId"]').val();
if (receiverAreaId) {
if (bannce(aeraNumArr[1],aeraNumArr[2],receiverAreaId)) {
setTimeout(function(){
self_btn_show();
}, 100);
};
};
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
$('.selfShipSubmit').removeAttr('disabled');
fubottom('提交失败,请重试');
}else if(status=='timeout'){
$('.selfShipSubmit').removeAttr('disabled');
fubottom('提交失败,请重试');
}
}
});
/*防止多次提交*/
$('.selfShipSubmit').attr('disabled', 'disabled');
setTimeout(function(){
$('.selfShipSubmit').removeAttr('disabled');
}, 5000);
}else{
$.MsgBox.Alert("地址获取失败,请重试或修改地址后再试",'确定');
}
});
})
}
});
})
}else{
AMap.service('AMap.Geocoder',function(){
loadingRemove();
var geocoder = new AMap.Geocoder({});
geocoder.getLocation(aeraArr[0]+""+aeraArr[1]+""+aeraArr[2]+""+detailed, function(status, result) {
if (status === 'complete' && result.info === 'OK1') {
loading('提交中...');
var data={
userId:userId(),
type:1,
id:senderAddId,
company:company,
contact:contact,
phone:phone,
proName:aeraArr[0],
proNumber:aeraNumArr[0],
cityName:aeraArr[1],
cityNumber:aeraNumArr[1],
areaName:aeraArr[2],
areaNumber:aeraNumArr[2],
detailed:detailed,
lat:result.geocodes[0].location.lat,
lng:result.geocodes[0].location.lng,
isDefault:isDefault,
requestTokenId:requestTokenId()
}
// console.log(data);
$.ajax({
url: apiL+'/api/wx/address/edit',
type: 'get',
timeout : 10000,
dataType: 'json',
data: data,
success:function(data){
loadingRemove();
if (data.success) {
// console.log(data)
$('[name="senderName"]').val(company);
$('[name="senderLinkman"]').val(contact);
$('[name="senderMobile"]').val(phone);
$('[name="senderProvinceName"]').val(aeraArr[0]);
$('[name="senderCityName"]').val(aeraArr[1]);
$('[name="senderAreaName"]').val(aeraArr[2]);
$('[name="senderProvinceId"]').val(aeraNumArr[0]);
$('[name="senderCityId"]').val(aeraNumArr[1]);
$('[name="senderAreaId"]').val(aeraNumArr[2]);
$('[name="senderAddress"]').val(detailed);
var html='<p id="addr_consigner"> '+company+' <br> '+contact+' '+phone+' <br>'+aeraArr[0]+' '+aeraArr[1]+' '+aeraArr[2]+' '+detailed+'</p>';
$('.consigner_edit a').html(html);
$('.self_fa_edit').remove();
var receiverAreaId=$('[name="receiverAreaId"]').val();
if (receiverAreaId) {
if (bannce(aeraNumArr[1],aeraNumArr[2],receiverAreaId)) {
setTimeout(function(){
self_btn_show();
}, 100);
};
};
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
$('.selfShipSubmit').removeAttr('disabled');
fubottom('提交失败,请重试');
}else if(status=='timeout'){
$('.selfShipSubmit').removeAttr('disabled');
fubottom('提交失败,请重试');
}
}
});
}else{
AMap.service('AMap.Geocoder',function(){//回调函数
//实例化Geocoder
var geocoder = new AMap.Geocoder({
// city: "010"//城市,默认:“全国”
});
geocoder.getLocation(aeraArr[0]+""+aeraArr[1]+""+aeraArr[2], function(status, result) {
loadingRemove();
if (status === 'complete' && result.info === 'OK') {
loading('提交中...');
var data={
userId:userId(),
type:1,
id:senderAddId,
company:company,
contact:contact,
phone:phone,
proName:aeraArr[0],
proNumber:aeraNumArr[0],
cityName:aeraArr[1],
cityNumber:aeraNumArr[1],
areaName:aeraArr[2],
areaNumber:aeraNumArr[2],
detailed:detailed,
lat:result.geocodes[0].location.lat,
lng:result.geocodes[0].location.lng,
isDefault:isDefault,
requestTokenId:requestTokenId()
}
// console.log(data);
$.ajax({
url: apiL+'/api/wx/address/edit',
type: 'get',
timeout : 10000,
dataType: 'json',
data: data,
success:function(data){
loadingRemove();
if (data.success) {
// console.log(data)
$('[name="senderName"]').val(company);
$('[name="senderLinkman"]').val(contact);
$('[name="senderMobile"]').val(phone);
$('[name="senderProvinceName"]').val(aeraArr[0]);
$('[name="senderCityName"]').val(aeraArr[1]);
$('[name="senderAreaName"]').val(aeraArr[2]);
$('[name="senderProvinceId"]').val(aeraNumArr[0]);
$('[name="senderCityId"]').val(aeraNumArr[1]);
$('[name="senderAreaId"]').val(aeraNumArr[2]);
$('[name="senderAddress"]').val(detailed);
var html='<p id="addr_consigner"> '+company+' <br> '+contact+' '+phone+' <br>'+aeraArr[0]+' '+aeraArr[1]+' '+aeraArr[2]+' '+detailed+'</p>';
$('.consigner_edit a').html(html);
$('.self_fa_edit').remove();
var receiverAreaId=$('[name="receiverAreaId"]').val();
if (receiverAreaId) {
if (bannce(aeraNumArr[1],aeraNumArr[2],receiverAreaId)) {
setTimeout(function(){
self_btn_show();
}, 100);
};
};
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
$('.selfShipSubmit').removeAttr('disabled');
fubottom('提交失败,请重试');
}else if(status=='timeout'){
$('.selfShipSubmit').removeAttr('disabled');
fubottom('提交失败,请重试');
}
}
});
}else{
$.MsgBox.Alert("地址获取失败,请重试或修改地址后再试",'确定');
}
});
})
}
});
})
};
}
}
}
/*显示发货添加*/
function selfConsigner(){
var html=template('self_fa_edit');
$('body').append(html);
$('.self_help_w').animate({right:0}, 300);
var senderName=$('[name="senderName"]').val();
var senderLinkman=$('[name="senderLinkman"]').val();
var senderMobile=$('[name="senderMobile"]').val();
var senderProvinceName=$('[name="senderProvinceName"]').val();
var senderCityName=$('[name="senderCityName"]').val();
var senderAreaName=$('[name="senderAreaName"]').val();
var senderProvinceId=$('[name="senderProvinceId"]').val();
var senderCityId=$('[name="senderCityId"]').val();
var senderAreaId=$('[name="senderAreaId"]').val();
var senderAddress=$('[name="senderAddress"]').val();
if (senderName) {
$('.consigner_t [name="client"]').val(senderName);
$('.consigner_t [name="name"]').val(senderLinkman);
$('.consigner_t [name="tel"]').val(senderMobile);
$('.consigner_t #addr_aera').html(senderProvinceName+' '+senderCityName+' '+senderAreaName).removeClass('c999');
$('.consigner_t [name="aeraNum"]').val(senderProvinceId+','+senderCityId+','+senderAreaId);
$('.consigner_t [name="detail"]').val(senderAddress);
addr_aera2(senderProvinceId,senderCityId,senderAreaId);
}else{
addr_aera2();
};
}
/*收货添加提交*/
function selfRedSubmit(){
if (!noNullfa($('[name="client"]'),'单位名称')) {
noNullfa($('[name="client"]'),'单位名称');
return false;
}else if(!telNo($('[name="tel"]'))){
telNo($('[name="tel"]'))
return false;
}else if(!noNull($('[name="aeraNum"]'),'所在省、市、区')){
noNull($('[name="aeraNum"]'),'所在省、市、区');
return false;
}else if(!noNullfa($('[name="detail"]'),'详细地址')){
noNullfa($('[name="detail"]'),'详细地址');
return false;
}else{
var company=trim($('[name="client"]').val());
var contact=trim($('[name="name"]').val());
var phone=trim($('[name="tel"]').val());
var aera=$('#addr_aera').html();
var aeraNum=$('[name="aeraNum"]').val();
var detailed=trim($('[name="detail"]').val());
var receiverRegisterCompany=$('[name="receiverRegisterCompany"]').val();
var receiverRegisterMobile=$('[name="receiverRegisterMobile"]').val();
var registerTelLin=$('[name="registerTelLin"]').val();
var registerConLin=$('[name="registerConLin"]').val();
var receiverIdLin=$('[name="receiverIdLin"]').val();
var aeraArr=aera.split(' ');
var aeraNumArr=aeraNum.split(',');
var isDefault=0;
if (senddistinctive()) {
$('[name="receiverRegisterCompany"]').val(company);
if (registerTelLin) {
if (registerConLin==company) {
$('[name="receiverRegisterMobile"]').val(registerTelLin);
}else{
$('[name="receiverRegisterMobile"]').val('');
}
}else{
$('[name="receiverRegisterMobile"]').val('');
};
/*检索的客户档案号*/
if (registerConLin==company) {
$('[name="receiverId"]').val(receiverIdLin);
}else{
$('[name="receiverId"]').val('');
};
$('[name="receiverName"]').val(company);
$('[name="receiverLinkman"]').val(contact);
$('[name="receiverMobile"]').val(phone);
$('[name="receiverProvinceName"]').val(aeraArr[0]);
$('[name="receiverCityName"]').val(aeraArr[1]);
$('[name="receiverAreaName"]').val(aeraArr[2]);
$('[name="receiverProvinceId"]').val(aeraNumArr[0]);
$('[name="receiverCityId"]').val(aeraNumArr[1]);
$('[name="receiverAreaId"]').val(aeraNumArr[2]);
$('[name="receiverAddress"]').val(detailed);
var html='<p id="addr_receiver"> '+company+' <br> '+contact+' '+phone+' <br>'+aeraArr[0]+' '+aeraArr[1]+' '+aeraArr[2]+' '+detailed+'</p>';
$('.delivery_edit a').html(html);
$('.self_shou_edit,.self_shou_add').remove();
$('.self_shou_edit').remove();
var senderCityId=$('[name="senderCityId"]').val();
var senderAreaId=$('[name="senderAreaId"]').val();
if (senderAreaId) {
if (bannce(senderCityId,senderAreaId,aeraNumArr[2])) {
setTimeout(function(){
self_btn_show();
}, 100);
};
};
};
}
}
/*发开添加编辑收货地址*/
function selfDelivery(){
var html=template('self_shou_add');
$('body').append(html);
$('.self_help_w').animate({right:0}, 300);
var receiverName=$('[name="receiverName"]').val();
var receiverLinkman=$('[name="receiverLinkman"]').val();
var receiverMobile=$('[name="receiverMobile"]').val();
var receiverProvinceName=$('[name="receiverProvinceName"]').val();
var receiverCityName=$('[name="receiverCityName"]').val();
var receiverAreaName=$('[name="receiverAreaName"]').val();
var receiverProvinceId=$('[name="receiverProvinceId"]').val();
var receiverCityId=$('[name="receiverCityId"]').val();
var receiverAreaId=$('[name="receiverAreaId"]').val();
var receiverAddress=$('[name="receiverAddress"]').val();
var receiverRegisterMobile=$('[name="receiverRegisterMobile"]').val();
var receiverRegisterCompany=$('[name="receiverRegisterCompany"]').val();
var receiverId=$('[name="receiverId"]').val();
if (receiverName) {
$('.consigner_t [name="client"]').val(receiverName);
$('.consigner_t [name="name"]').val(receiverLinkman);
$('.consigner_t [name="tel"]').val(receiverMobile);
$('.consigner_t #addr_aera').html(receiverProvinceName+' '+receiverCityName+' '+receiverAreaName).removeClass('c999');
$('.consigner_t [name="aeraNum"]').val(receiverProvinceId+','+receiverCityId+','+receiverAreaId);
$('.consigner_t [name="detail"]').val(receiverAddress);
$('.consigner_t [name="registerTelLin"]').val(receiverRegisterMobile);
$('.consigner_t [name="registerConLin"]').val(receiverRegisterCompany);
$('.consigner_t [name="receiverIdLin"]').val(receiverId);
addr_aera2(receiverProvinceId,receiverCityId,receiverAreaId);
}else{
addr_aera2();
};
}
/*发货地址内容(自助下单)*/
function selfshipAddr(data){
var html="";
for (var i = 0; i < data.info.length; i++) {
if (data.info[i].isDefault) {
html+='<div class="record_li ship_li" data-id="'+data.info[i].id+'">'
+'<table width="w100" onclick=addrTrue('+data.info[i].id+')>'
+'<col width="74%"><col width="20%">'
+'<tr><td>发 货 方:'+data.info[i].company+'</td><td class="t_r moren"><span class="red">默认发货</span></td></tr>'
if (data.info[i].contact) {
html+='<tr><td colspan="2">联 系 人:'+data.info[i].contact+'</td></tr>'
};
html+='<tr><td colspan="2">电  话:'+data.info[i].phone+'<br></td></tr>'
+'<tr><td colspan="2">发货地址:'+data.info[i].proName+''+data.info[i].cityName+''+data.info[i].areaName+''+data.info[i].detailed+'<br></td></tr></table>'
+'<div class="show_details ov_h">'
+'<label class="ship_moren"></label>'
+'<a class="f_r f14" href="javascript:;" onclick="del_addr($(this),'+data.info[i].id+')">删除</a>'
+'<a class="f_r f14" onclick="selfConsignerEdit('+data.info[i].id+')" href="javascript:;">编辑</a>'
+'</div>'
+'</div>'
}else{
html+='<div class="record_li ship_li" data-id="'+data.info[i].id+'">'
+'<table width="w100" onclick=addrTrue('+data.info[i].id+')>'
+'<col width="74%"><col width="20%">'
+'<tr><td>发 货 方:'+data.info[i].company+'</td><td class="t_r moren"></td></tr>'
if (data.info[i].contact) {
html+='<tr><td colspan="2">联 系 人:'+data.info[i].contact+'</td></tr>'
};
html+='<tr><td colspan="2">电  话:'+data.info[i].phone+'<br></td></tr>'
+'<tr><td colspan="2">发货地址:'+data.info[i].proName+''+data.info[i].cityName+''+data.info[i].areaName+''+data.info[i].detailed+'<br></td></tr></table>'
+'</table>'
+'<div class="show_details ov_h">'
+'<label class="ship_moren"><input type="radio" name="default"><span class="radio"></span>设置为默认发货方</label>'
+'<a class="f_r f14" href="javascript:;" onclick="del_addr($(this),'+data.info[i].id+')">删除</a>'
+'<a class="f_r f14" onclick="selfConsignerEdit('+data.info[i].id+')" href="javascript:;">编辑</a>'
+'</div>'
+'</div>'
};
};
$('.record_list').html(html);
}
/*发货地址薄搜索(自助下单)*/
function selfAddrsearch(){
if (userId()) {
loading('loading...');
var search=$('.search_input').val();
var data={
userId:userId(),
type:1,
searchParam:search,
requestTokenId:requestTokenId()
}
if (search){
$.ajax({
url: api+'/api/wx/address/find',
type: 'get',
timeout : 10000,
dataType: 'json',
data: data,
success:function(data){
if (data.success) {
if (data.info.length) {
selfshipAddr(data)
}else{
noshipsearch(search);
};
}else{
alert(data.message)
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','selfAddrsearch()');
}else if(status=='timeout'){
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','selfAddrsearch()');
}
}
});
}else{
$.ajax({
url: apiL+'/api/wx/address/find',
type: 'get',
timeout : 10000,
dataType: 'json',
data: {userId:userId(),type:1,requestTokenId:requestTokenId()},
success:function(data){
if (data.success) {
if (data.info.length) {
selfshipAddr(data)
}else{
noshipsearch();
}
}else{
alert(data.message)
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','selfAddrsearch()');
}else if(status=='timeout'){
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','selfAddrsearch()');
}
}
});
};
}else{
document.write('请求失败,请返回公众号重新进入');
}
}
/*以上为发货地址薄*/
/*发货方地址列表*/
function selfConsignerBook(){
var html=template('self_fa_book');
$('body').append(html);
$('.self_help_w').animate({right:0}, 300);
if (userId()) {
selfConsignerBookA();
}else{
document.write('请求失败,请返回公众号重新进入');
}
}
function selfConsignerBookA(){
loading('loading...');
$.ajax({
url: apiL+'/api/wx/address/find',
type: 'get',
timeout : 10000,
dataType: 'json',
data: {userId:userId(),type:1,requestTokenId:requestTokenId()},
success:function(data){
if (data.success) {
if (data.info.length) {
selfshipAddr(data);
}else{
noshipsearch();
};
}else{
alert(data.message)
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','selfConsignerBookA()');
}else if(status=='timeout'){
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','selfConsignerBookA()');
}
}
});
}
/*没有地址列表*/
function noshipsearch(name){
if (name) {
$('.record_list').html('<div class="ship_nofind"><span><img src="../skin/images/no_find.png" alt=""></span><p>对不起,没有找到“'+name+'”,请重新输入</p></div>')
}else{
$('.record_list').html('<div class="ship_nofind"><span><img src="../skin/images/no_find.png" alt=""></span><p>对不起,您还没有添加地址</p></div>')
};
}
/*列表选择发货方*/
function addrTrue(id){
loading('loading...');
$.ajax({
url: apiL+'/api/wx/address/findedit',
type: 'get',
timeout : 10000,
dataType: 'json',
data: {type:1,id:id,requestTokenId:requestTokenId()},
success:function(data){
loadingRemove();
if (data.success) {
var info=data.info;
if (info.company==$('[name="receiverName"]').val()) {
$.MsgBox.Alert("发货方与收货方名称不能相同",'确定');
return false;
};
/*if (info.phone==$('[name="receiverMobile"]').val()) {
$.MsgBox.Alert("发货方电话不能与收货方电话相同",'确定');
return false;
};*/
if (info.contact) {
var contact=info.contact;
}else{
var contact='';
};
$('[name="senderAddId"]').val(id);
$('[name="senderAddIsDf"]').val(info.isDefault);
$('[name="senderName"]').val(info.company);
$('[name="senderLinkman"]').val(contact);
$('[name="senderMobile"]').val(info.phone);
$('[name="senderProvinceName"]').val(info.proName);
$('[name="senderCityName"]').val(info.cityName);
$('[name="senderAreaName"]').val(info.areaName);
$('[name="senderProvinceId"]').val(info.proNumber);
$('[name="senderCityId"]').val(info.cityNumber);
$('[name="senderAreaId"]').val(info.areaNumber);
$('[name="senderAddress"]').val(info.detailed);
var html='<p id="addr_consigner"> '+info.company+' <br> '+contact+' '+info.phone+' <br>'+info.proName+' '+info.cityName+' '+info.areaName+' '+info.detailed+'</p>';
$('.consigner_edit a').html(html);
$('.self_fa_book').remove();
var receiverAreaId=$('[name="receiverAreaId"]').val();
if (receiverAreaId) {
if (bannce(info.cityNumber,info.areaNumber,receiverAreaId)) {
setTimeout(function(){
self_btn_show();
}, 100);
};
};
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
fubottom('选取失败,请重试');
}else if(status=='timeout'){
fubottom('选取失败,请重试');
}
}
});
}
/*选择列表选择收货方*/
function recTrue(id){
loading('loading...');
$.ajax({
url: apiL+'/api/wx/address/findedit',
type: 'get',
timeout : 10000,
dataType: 'json',
data: {type:2,id:id,requestTokenId:requestTokenId()},
success:function(data){
loadingRemove();
if (data.success) {
var info=data.info;
if (info.company==$('[name="senderName"]').val()) {
$.MsgBox.Alert("收货方与发货方单位名称不能相同",'确定');
return false;
};
/*if (info.phone==$('[name="senderMobile"]').val()) {
$.MsgBox.Alert("收货方电话不能与发货方电话相同",'确定');
return false;
};*/
$('[name="receiverName"]').val(info.company);
$('[name="receiverLinkman"]').val(info.contact);
$('[name="receiverMobile"]').val(info.phone);
$('[name="receiverProvinceName"]').val(info.proName);
$('[name="receiverCityName"]').val(info.cityName);
$('[name="receiverAreaName"]').val(info.areaName);
$('[name="receiverProvinceId"]').val(info.proNumber);
$('[name="receiverCityId"]').val(info.cityNumber);
$('[name="receiverAreaId"]').val(info.areaNumber);
$('[name="receiverAddress"]').val(info.detailed);
$('[name="receiverRegisterMobile"]').val('');
$('[name="receiverRegisterCompany"]').val('');
$('[name="receiverId"]').val('');
var html='<p id="addr_receiver"> '+info.company+' <br> ';
if (info.contact) {
html+=info.contact+' ';
};
html+=info.phone+' <br>'+info.proName+' '+info.cityName+' '+info.areaName+' '+info.detailed+'</p>';
$('.delivery_edit a').html(html);
$('.self_shou_book').remove();
/*获取网点*/
var senderCityId=$('[name="senderCityId"]').val();
var senderAreaId=$('[name="senderAreaId"]').val();
if (senderAreaId) {
if (bannce(senderCityId,senderAreaId,info.areaNumber)) {
setTimeout(function(){
self_btn_show();
}, 100);
};
};
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
fubottom('选取失败,请重试');
}else if(status=='timeout'){
fubottom('选取失败,请重试');
}
}
});
}
/*发货地址簿删除*/
function del_addr(e,id){
$.MsgBox.ConfirmL("确定要删除吗?",'取消','确定',function(){
loading('删除中...');
$.ajax({
url: apiL+'/api/wx/address/findedit',
type: 'post',
timeout : 10000,
dataType: 'json',
data: {id:id,type:1,requestTokenId:requestTokenId()},
success:function(data){
loadingRemove();
var isDefault=data.info.isDefault;
if (data.success) {
$.ajax({
url: apiL+'/api/wx/address/delete',
type: 'post',
timeout : 10000,
dataType: 'json',
data: {type:1,id:id,userId:userId(),isDefault:isDefault,requestTokenId:requestTokenId()},
success:function(data1){
if (data1.success) {
$.ajax({
url: apiL+'/api/wx/address/find',
type: 'get',
dataType: 'json',
data: {userId:userId(),type:1,requestTokenId:requestTokenId()},
success:function(data){
if (data.success) {
var html="";
for (var i = 0; i < data.info.length; i++) {
if (data.info[i].isDefault==1) {
html='<p id="addr_consigner"> '+data.info[i].company+' <br> '+data.info[i].contact+' '+data.info[i].phone+' <br>'+data.info[i].proName+' '+data.info[i].cityName+' '+data.info[i].areaName+' '+data.info[i].detailed+'</p>';
$('.consigner_edit a').html(html);
$('[name="senderAddId"]').val(data.info[i].id);
$('[name="senderAddIsDf"]').val(data.info[i].isDefault);
$('[name="senderName"]').val(data.info[i].company);
$('[name="senderLinkman"]').val(data.info[i].contact);
$('[name="senderMobile"]').val(data.info[i].phone);
$('[name="senderProvinceName"]').val(data.info[i].proName);
$('[name="senderCityName"]').val(data.info[i].cityName);
$('[name="senderAreaName"]').val(data.info[i].areaName);
$('[name="senderProvinceId"]').val(data.info[i].proNumber);
$('[name="senderCityId"]').val(data.info[i].cityNumber);
$('[name="senderAreaId"]').val(data.info[i].areaNumber);
$('[name="senderAddress"]').val(data.info[i].detailed);
/*默认网点*/
var receiverAreaId=$('[name="receiverAreaId"]').val();
if (receiverAreaId) {
bannce(data.info[i].cityNumber,data.info[i].areaNumber,receiverAreaId)
};
};
};
if (data.info.length==0) {
$('[name="senderAddId"]').val('');
$('[name="senderAddIsDf"]').val('');
};
if (data.info.length) {
selfshipAddr(data);
}else{
noshipsearch();
}
}else{
alert(data.message)
};
}
});
}else{
alert(data1.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
fubottom('删除失败,请重试');
}else if(status=='timeout'){
fubottom('删除失败,请重试');
}
}
});
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
fubottom('删除失败,请重试');
}else if(status=='timeout'){
fubottom('删除失败,请重试');
}
}
});
},function(){
return false;
})
};
/*收地址簿删除*/
function del_rec(e,id){
$.MsgBox.ConfirmL("确定要删除吗?",'取消','确定',function(){
loading('删除中...');
$.ajax({
url: apiL+'/api/wx/address/delete',
type: 'post',
timeout : 10000,
dataType: 'json',
data: {type:2,id:id,userId:userId(),isDefault:0,requestTokenId:requestTokenId()},
success:function(data){
loadingRemove();
if (data.success) {
e.parents('.record_li').remove();
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
fubottom('删除失败,请重试');
}else if(status=='timeout'){
fubottom('删除失败,请重试');
}
}
});
},function(){
return false;
})
};
/*编辑发货列表地址获取*/
function selfConsignerEdit(id){
/*$('.self_fa_book').remove();*/
var html=template('self_fa_edit');
$('body').append(html);
$('.self_help_w').animate({right:0}, 300);
$('.selfShipSubmit').attr('onclick', 'selfShipEditSubmit('+id+')');
$('.tacitly_approve').html('<label><input type="checkbox" name="default"><span class="checkbox"></span><b id="">设为默认发货方</b></label>');
loading('获取中...');
$.ajax({
url: apiL+'/api/wx/address/findedit',
type: 'get',
timeout : 10000,
dataType: 'json',
data: {type:1,id:id,requestTokenId:requestTokenId()},
success:function(data){
if (data.success) {
var info=data.info;
$('.consigner_t [name="client"]').val(info.company);
$('.consigner_t [name="name"]').val(info.contact);
$('.consigner_t [name="tel"]').val(info.phone);
$('.consigner_t #addr_aera').html(info.proName+' '+info.cityName+' '+info.areaName).removeClass('c999');
$('.consigner_t [name="aeraNum"]').val(info.proNumber+','+info.cityNumber+','+info.areaNumber);
$('.consigner_t [name="detail"]').val(info.detailed);
addr_aera2(info.proNumber,info.cityNumber,info.areaNumber);
if (info.isDefault) {
$('[name="default"]').attr('checked', 'checked');
};
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
$.MsgBox.Alert("获取信息失败,请点击关闭重试",'好的');
}else if(status=='timeout'){
$.MsgBox.Alert("获取信息失败,请点击关闭重试",'好的');
}
}
});
}
/*编辑发货列表地址提交*/
function selfShipEditSubmit(id){
if (!noNullfa($('[name="client"]'),'单位名称')) {
noNullfa($('[name="client"]'),'单位名称');
return false;
}else if(!nameLen($('[name="name"]').val())){
nameLen($('[name="name"]').val());
return false;
}else if(!telNo($('[name="tel"]'))){
telNo($('[name="tel"]'))
return false;
}else if(!noNull($('[name="aeraNum"]'),'所在省、市、区')){
noNull($('[name="aeraNum"]'),'所在省、市、区');
return false;
}else if(!noNullfa($('[name="detail"]'),'详细地址')){
noNullfa($('[name="detail"]'),'详细地址');
return false;
}else{
loading('获取中...');
var company=trim($('[name="client"]').val());
var contact=trim($('[name="name"]').val());
var phone=trim($('[name="tel"]').val());
var aera=$('#addr_aera').html();
var aeraNum=$('[name="aeraNum"]').val();
var detailed=trim($('[name="detail"]').val());
var aeraArr=aera.split(' ');
var aeraNumArr=aeraNum.split(',');
var isDefault="";
if ($('[name="default"]:checked').length>0) {
isDefault=1;
}else{
isDefault=0;
};
AMap.service('AMap.Geocoder',function(){
var geocoder = new AMap.Geocoder({});
geocoder.getLocation(aeraArr[0]+""+aeraArr[1]+""+aeraArr[2]+""+detailed, function(status, result) {
loadingRemove();
if (status === 'complete' && result.info === 'OK') {
loading('获取中...');
var data={
userId:userId(),
type:1,
id:id,
company:company,
contact:contact,
phone:phone,
proName:aeraArr[0],
proNumber:aeraNumArr[0],
cityName:aeraArr[1],
cityNumber:aeraNumArr[1],
areaName:aeraArr[2],
areaNumber:aeraNumArr[2],
detailed:detailed,
lat:result.geocodes[0].location.lat,
lng:result.geocodes[0].location.lng,
isDefault:isDefault,
requestTokenId:requestTokenId()
}
$.ajax({
url: apiL+'/api/wx/address/edit',
type: 'get',
timeout : 10000,
dataType: 'json',
data: data,
success:function(data){
loadingRemove();
if (data.success) {
if ($('[name="senderAddId"]').val()==id) {
if(Recdistinctive()){
$.ajax({
url: apiL+'/api/wx/address/findedit',
type: 'get',
timeout : 10000,
dataType: 'json',
data: {type:1,id:id,requestTokenId:requestTokenId()},
success:function(data){
if (data.success) {
var info=data.info;
$('[name="senderAddIsDf"]').val(info.isDefault);
$('[name="senderName"]').val(info.company);
$('[name="senderLinkman"]').val(info.contact);
$('[name="senderMobile"]').val(info.phone);
$('[name="senderProvinceName"]').val(info.proName);
$('[name="senderCityName"]').val(info.cityName);
$('[name="senderAreaName"]').val(info.areaName);
$('[name="senderProvinceId"]').val(info.proNumber);
$('[name="senderCityId"]').val(info.cityNumber);
$('[name="senderAreaId"]').val(info.areaNumber);
$('[name="senderAddress"]').val(info.detailed);
var contact="";
info.contact?contact=info.contact:contact='';
var html='<p id="addr_consigner"> '+info.company+' <br> '+contact+' '+info.phone+' <br>'+info.proName+' '+info.cityName+' '+info.areaName+' '+info.detailed+'</p>';
$('.consigner_edit a').html(html);
var receiverAreaId=$('[name="receiverAreaId"]').val();
if (receiverAreaId) {
if (bannce(info.cityNumber,info.areaNumber,receiverAreaId)) {
setTimeout(function(){
self_btn_show();
}, 100);
};
};
$('.self_fa_edit').remove();
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
fubottom('保存失败,请重试');
}else if(status=='timeout'){
fubottom('保存失败,请重试');
}
return false;
}
});
$.ajax({
url: apiL+'/api/wx/address/find',
type: 'get',
timeout : 10000,
dataType: 'json',
data: {userId:userId(),type:1,requestTokenId:requestTokenId()},
success:function(data){
if (data.success) {
if (data.info.length) {
selfshipAddr(data);
}else{
noshipsearch();
};
}else{
alert(data.message)
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
noResultErr($('.record_list'),400,'获取列表失败,请点击重新获取','selfConsignerBookA()');
}else if(status=='timeout'){
noResultErr($('.record_list'),400,'获取列表失败,请点击重新获取','selfConsignerBookA()');
}
}
});
}
}else{
$('.self_fa_edit').remove();
$.ajax({
url: apiL+'/api/wx/address/find',
type: 'get',
timeout : 10000,
dataType: 'json',
data: {userId:userId(),type:1,requestTokenId:requestTokenId()},
success:function(data){
if (data.success) {
if (data.info.length) {
selfshipAddr(data);
}else{
noshipsearch();
};
}else{
alert(data.message)
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
noResultErr($('.record_list'),400,'获取列表失败,请点击重新获取','selfConsignerBookA()');
}else if(status=='timeout'){
noResultErr($('.record_list'),400,'获取列表失败,请点击重新获取','selfConsignerBookA()');
}
}
});
}
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
fubottom('保存失败,请重试');
}else if(status=='timeout'){
fubottom('保存失败,请重试');
}
}
});
}else{
AMap.service('AMap.Geocoder',function(){//回调函数
//实例化Geocoder
var geocoder = new AMap.Geocoder({
// city: "010"//城市,默认:“全国”
});
geocoder.getLocation(aeraArr[0]+""+aeraArr[1]+""+aeraArr[2], function(status, result) {
loadingRemove();
if (status === 'complete' && result.info === 'OK') {
loading('获取中...');
var data={
userId:userId(),
type:1,
id:id,
company:company,
contact:contact,
phone:phone,
proName:aeraArr[0],
proNumber:aeraNumArr[0],
cityName:aeraArr[1],
cityNumber:aeraNumArr[1],
areaName:aeraArr[2],
areaNumber:aeraNumArr[2],
detailed:detailed,
lat:result.geocodes[0].location.lat,
lng:result.geocodes[0].location.lng,
isDefault:isDefault,
requestTokenId:requestTokenId()
}
$.ajax({
url: apiL+'/api/wx/address/edit',
type: 'get',
timeout : 10000,
dataType: 'json',
data: data,
success:function(data){
loadingRemove();
if (data.success) {
if ($('[name="senderAddId"]').val()==id) {
if(Recdistinctive()){
$.ajax({
url: apiL+'/api/wx/address/findedit',
type: 'get',
timeout : 10000,
dataType: 'json',
data: {type:1,id:id,requestTokenId:requestTokenId()},
success:function(data){
if (data.success) {
var info=data.info;
$('[name="senderAddIsDf"]').val(info.isDefault);
$('[name="senderName"]').val(info.company);
$('[name="senderLinkman"]').val(info.contact);
$('[name="senderMobile"]').val(info.phone);
$('[name="senderProvinceName"]').val(info.proName);
$('[name="senderCityName"]').val(info.cityName);
$('[name="senderAreaName"]').val(info.areaName);
$('[name="senderProvinceId"]').val(info.proNumber);
$('[name="senderCityId"]').val(info.cityNumber);
$('[name="senderAreaId"]').val(info.areaNumber);
$('[name="senderAddress"]').val(info.detailed);
var contact="";
info.contact?contact=info.contact:contact='';
var html='<p id="addr_consigner"> '+info.company+' <br> '+contact+' '+info.phone+' <br>'+info.proName+' '+info.cityName+' '+info.areaName+' '+info.detailed+'</p>';
$('.consigner_edit a').html(html);
var receiverAreaId=$('[name="receiverAreaId"]').val();
if (receiverAreaId) {
if (bannce(info.cityNumber,info.areaNumber,receiverAreaId)) {
setTimeout(function(){
self_btn_show();
}, 100);
};
};
$('.self_fa_edit').remove();
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
fubottom('保存失败,请重试');
}else if(status=='timeout'){
fubottom('保存失败,请重试');
}
return false;
}
});
$.ajax({
url: apiL+'/api/wx/address/find',
type: 'get',
timeout : 10000,
dataType: 'json',
data: {userId:userId(),type:1,requestTokenId:requestTokenId()},
success:function(data){
if (data.success) {
if (data.info.length) {
selfshipAddr(data);
}else{
noshipsearch();
};
}else{
alert(data.message)
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
noResultErr($('.record_list'),400,'获取列表失败,请点击重新获取','selfConsignerBookA()');
}else if(status=='timeout'){
noResultErr($('.record_list'),400,'获取列表失败,请点击重新获取','selfConsignerBookA()');
}
}
});
}
}else{
$('.self_fa_edit').remove();
$.ajax({
url: apiL+'/api/wx/address/find',
type: 'get',
timeout : 10000,
dataType: 'json',
data: {userId:userId(),type:1,requestTokenId:requestTokenId()},
success:function(data){
if (data.success) {
if (data.info.length) {
selfshipAddr(data);
}else{
noshipsearch();
};
}else{
alert(data.message)
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
noResultErr($('.record_list'),400,'获取列表失败,请点击重新获取','selfConsignerBookA()');
}else if(status=='timeout'){
noResultErr($('.record_list'),400,'获取列表失败,请点击重新获取','selfConsignerBookA()');
}
}
});
}
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
fubottom('保存失败,请重试');
}else if(status=='timeout'){
fubottom('保存失败,请重试');
}
}
});
}else{
$.MsgBox.Alert("地址获取失败,请重试或修改地址后再试",'确定');
}
});
})
}
});
})
}
}
/*添加发货地址*/
function addFaEdit(){
var html=template('self_fa_edit');
$('body').append(html);
$('.self_help_w').animate({right:0}, 300);
/*$('.tacitly_approve').html('<label><input type="checkbox" name="default"><span class="checkbox"></span><b id="">设为默认收货方</b></label>')*/
$('.selfShipSubmit').attr('onclick', 'selfAddShipSubmit()');
addr_aera2();
}
/*添加发货地址列表提交*/
function selfAddShipSubmit(){
if (!noNullfa($('[name="client"]'),'单位名称')) {
noNullfa($('[name="client"]'),'单位名称');
return false;
}else if(!telNo($('[name="tel"]'))){
telNo($('[name="tel"]'))
return false;
}else if(!noNull($('[name="aeraNum"]'),'所在省、市、区')){
noNull($('[name="aeraNum"]'),'所在省、市、区');
return false;
}else if(!noNullfa($('[name="detail"]'),'详细地址')){
noNullfa($('[name="detail"]'),'详细地址');
return false;
}else{
loading('提交中...');
var company=trim($('[name="client"]').val());
var contact=trim($('[name="name"]').val());
var phone=trim($('[name="tel"]').val());
var aera=$('#addr_aera').html();
var aeraNum=$('[name="aeraNum"]').val();
var detailed=trim($('[name="detail"]').val());
var aeraArr=aera.split(' ');
var aeraNumArr=aeraNum.split(',');
var isDefault=0;
if ($('[name="default"]:checked').length>0) {
isDefault=1;
}else{
isDefault=0;
};
AMap.service('AMap.Geocoder',function(){
var geocoder = new AMap.Geocoder({});
geocoder.getLocation(aeraArr[0]+""+aeraArr[1]+""+aeraArr[2]+""+detailed, function(status, result) {
loadingRemove();
if (status === 'complete' && result.info === 'OK') {
loading('提交中...');
var data={
userId:userId(),
type:1,
company:company,
contact:contact,
phone:phone,
proName:aeraArr[0],
proNumber:aeraNumArr[0],
cityName:aeraArr[1],
cityNumber:aeraNumArr[1],
areaName:aeraArr[2],
areaNumber:aeraNumArr[2],
detailed:detailed,
lat:result.geocodes[0].location.lat,
lng:result.geocodes[0].location.lng,
isDefault:isDefault,
requestTokenId:requestTokenId()
};
$.ajax({
url: apiL+'/api/wx/address/add',
type: 'get',
timeout : 10000,
dataType: 'json',
data: data,
success:function(data){
loadingRemove();
if (data.success) {
$('.self_fa_edit').remove();
$.ajax({
url: apiL+'/api/wx/address/find',
type: 'get',
timeout : 10000,
dataType: 'json',
data: {userId:userId(),type:1,requestTokenId:requestTokenId()},
success:function(data){
if (data.success) {
if (data.info.length) {
selfshipAddr(data);
}else{
noshipsearch();
};
}else{
alert(data.message)
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
noResultErr($('.record_list'),400,'获取列表失败,请点击重新获取','selfConsignerBookA()');
}else if(status=='timeout'){
noResultErr($('.record_list'),400,'获取列表失败,请点击重新获取','selfConsignerBookA()');
}
}
});
/*selfConsignerBook();*/
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
fubottom('保存失败,请5秒后重试');
}else if(status=='timeout'){
fubottom('保存失败,请5秒后重试');
}
}
});
}else{
AMap.service('AMap.Geocoder',function(){//回调函数
//实例化Geocoder
var geocoder = new AMap.Geocoder({
// city: "010"//城市,默认:“全国”
});
geocoder.getLocation(aeraArr[0]+""+aeraArr[1]+""+aeraArr[2], function(status, result) {
loadingRemove();
if (status === 'complete' && result.info === 'OK') {
loading('提交中...');
var data={
userId:userId(),
type:1,
company:company,
contact:contact,
phone:phone,
proName:aeraArr[0],
proNumber:aeraNumArr[0],
cityName:aeraArr[1],
cityNumber:aeraNumArr[1],
areaName:aeraArr[2],
areaNumber:aeraNumArr[2],
detailed:detailed,
lat:result.geocodes[0].location.lat,
lng:result.geocodes[0].location.lng,
isDefault:isDefault,
requestTokenId:requestTokenId()
};
$.ajax({
url: apiL+'/api/wx/address/add',
type: 'get',
timeout : 10000,
dataType: 'json',
data: data,
success:function(data){
loadingRemove();
if (data.success) {
$('.self_fa_edit').remove();
$.ajax({
url: apiL+'/api/wx/address/find',
type: 'get',
timeout : 10000,
dataType: 'json',
data: {userId:userId(),type:1,requestTokenId:requestTokenId()},
success:function(data){
if (data.success) {
if (data.info.length) {
selfshipAddr(data);
}else{
noshipsearch();
};
}else{
alert(data.message)
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
noResultErr($('.record_list'),400,'获取列表失败,请点击重新获取','selfConsignerBookA()');
}else if(status=='timeout'){
noResultErr($('.record_list'),400,'获取列表失败,请点击重新获取','selfConsignerBookA()');
}
}
});
/*selfConsignerBook();*/
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
fubottom('保存失败,请5秒后重试');
}else if(status=='timeout'){
fubottom('保存失败,请5秒后重试');
}
}
});
}else{
$.MsgBox.Alert("地址获取失败,请重试或修改地址后再试",'确定');
}
});
})
}
});
})
$('.selfShipSubmit').attr('disabled', 'disabled').css('background', '#999');
setTimeout(function(){
$('.selfShipSubmit').removeAttr('disabled').css('background', '#52BB03');
}, 5000);
}
}
/*收货方地址列表*/
function selfDeliveryBook(){
var html=template('self_shou_book');
$('body').append(html);
$('.self_help_w').animate({right:0}, 300);
if (userId()) {
selfDeliveryBookA();
}else{
document.write('请求失败,请返回公众号重新进入');
};
}
function selfDeliveryBookA(){
loading('loading...');
$.ajax({
url: apiL+'/api/wx/address/find',
type: 'get',
timeout : 10000,
dataType: 'json',
data: {userId:userId(),type:2,requestTokenId:requestTokenId()},
success:function(data){
if (data.success) {
if (data.info.length) {
selfReceAddr(data);
}else{
noshipsearch();
};
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','selfDeliveryBookA()');
}else if(status=='timeout'){
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','selfDeliveryBookA()');
}
}
});
}
/*收货地址搜索(自助下单)*/
function selfAddrShouSearch(){
var search=$('.search_input').val();
loading('loading...');
if (search){
var data={
userId:userId(),
type:2,
searchParam:search,
requestTokenId:requestTokenId()
};
$.ajax({
url: api+'/api/wx/address/find',
type: 'get',
timeout : 10000,
dataType: 'json',
data: data,
success:function(data){
loadingRemove();
if (data.success) {
if (data.info.length) {
selfReceAddr(data);
}else{
noshipsearch(search);
};
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
noResultErr($('.record_list'),400,'获取列表失败,请点击重新获取','selfAddrShouSearch()');
}else if(status=='timeout'){
noResultErr($('.record_list'),400,'获取列表失败,请点击重新获取','selfAddrShouSearch()');
}
}
});
}else{
$.ajax({
url: apiL+'/api/wx/address/find',
type: 'get',
timeout : 10000,
dataType: 'json',
data: {userId:userId(),type:2,requestTokenId:requestTokenId()},
success:function(data){
loadingRemove();
if (data.success) {
if (data.info.length) {
selfReceAddr(data);
}else{
noshipsearch();
}
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
noResultErr($('.record_list'),400,'获取列表失败,请点击重新获取','selfAddrShouSearch()');
}else if(status=='timeout'){
noResultErr($('.record_list'),400,'获取列表失败,请点击重新获取','selfAddrShouSearch()');
}
}
});
};
}
/*收货地址内容(自助下单)*/
function selfReceAddr(data){
var html="";
for (var i = 0; i < data.info.length; i++) {
html+='<div class="record_li ship_li" data-id="'+data.info[i].id+'">'
+'<table width="w100" onclick=recTrue("'+data.info[i].id+'")>'
+'<tr><td>收 货 方:'+data.info[i].company+'</td></tr>'
if (data.info[i].contact) {
html+='<tr><td colspan="2">联 系 人:'+data.info[i].contact+'</td></tr>'
};
html+='<tr><td colspan="2">电  话:'+data.info[i].phone+'<br></td></tr>'
+'<tr><td colspan="2">收货地址:'+data.info[i].proName+''+data.info[i].cityName+''+data.info[i].areaName+''+data.info[i].detailed+'<br></td></tr>'
+'</table>'
+'<div class="show_details ov_h">'
+'<a class="f_r f14" href="javascript:;" onclick="del_rec($(this),'+data.info[i].id+')">删除</a>'
+'<a class="f_r f14" onclick="selfrecrivingEdit('+data.info[i].id+')" href="javascript:;">编辑</a>'
+'</div>'
+'</div>'
};
$('.record_list').html(html);
}
/*编辑收货列表地址获取*/
function selfrecrivingEdit(id){
/*$('.self_shou_book').remove();*/
var html=template('self_shou_edit');
$('body').append(html);
$('.self_help_w').animate({right:0}, 300);
/*$('.tacitly_approve').remove();*/
$('.selfRedSubmit').attr('onclick', 'selfRedEditSubmit('+id+')');
$.ajax({
url: apiL+'/api/wx/address/findedit',
type: 'get',
timeout : 10000,
dataType: 'json',
data: {type:2,id:id,requestTokenId:requestTokenId()},
success:function(data){
if (data.success) {
var info=data.info;
// console.log(info)
$('.consigner_t [name="client"]').val(info.company);
$('.consigner_t [name="name"]').val(info.contact);
$('.consigner_t [name="tel"]').val(info.phone);
$('.consigner_t #addr_aera').html(info.proName+' '+info.cityName+' '+info.areaName).removeClass('c999');
$('.consigner_t [name="aeraNum"]').val(info.proNumber+','+info.cityNumber+','+info.areaNumber);
$('.consigner_t [name="detail"]').val(info.detailed);
addr_aera2(info.proNumber,info.cityNumber,info.areaNumber);
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
$.MsgBox.Alert("获取信息失败,请点击关闭重试",'好的');
}else if(status=='timeout'){
$.MsgBox.Alert("获取信息失败,请点击关闭重试",'好的');
}
}
});
}
/*编辑收货列表地址提交*/
function selfRedEditSubmit(id){
if (!noNullfa($('[name="client"]'),'单位名称')) {
noNullfa($('[name="client"]'),'单位名称');
return false;
}else if(!nameLen($('[name="name"]').val())){
nameLen($('[name="name"]').val());
return false;
}else if(!telNo($('[name="tel"]'))){
telNo($('[name="tel"]'));
return false;
}else if(!noNull($('[name="aeraNum"]'),'所在省、市、区')){
noNull($('[name="aeraNum"]'),'所在省、市、区');
return false;
}else if(!noNullfa($('[name="detail"]'),'详细地址')){
noNullfa($('[name="detail"]'),'详细地址');
return false;
}else{
loading('提交中...');
var company=trim($('[name="client"]').val());
var contact=trim($('[name="name"]').val());
var phone=trim($('[name="tel"]').val());
var aera=$('#addr_aera').html();
var aeraNum=$('[name="aeraNum"]').val();
var detailed=trim($('[name="detail"]').val());
var aeraArr=aera.split(' ');
var aeraNumArr=aeraNum.split(',');
AMap.service('AMap.Geocoder',function(){
var geocoder = new AMap.Geocoder({});
geocoder.getLocation(aeraArr[0]+""+aeraArr[1]+""+aeraArr[2]+""+detailed, function(status, result) {
loadingRemove();
if (status === 'complete' && result.info === 'OK') {
loading('提交中...');
var data={
userId:userId(),
type:2,
id:id,
company:company,
contact:contact,
phone:phone,
proName:aeraArr[0],
proNumber:aeraNumArr[0],
cityName:aeraArr[1],
cityNumber:aeraNumArr[1],
areaName:aeraArr[2],
areaNumber:aeraNumArr[2],
detailed:detailed,
lat:result.geocodes[0].location.lat,
lng:result.geocodes[0].location.lng,
isDefault:0,
requestTokenId:requestTokenId()
}
$.ajax({
url: apiL+'/api/wx/address/edit',
type: 'get',
timeout : 10000,
dataType: 'json',
data: data,
success:function(data){
loadingRemove();
if (data.success) {
$('.self_shou_edit').remove();
/*selfDeliveryBook();*/
$.ajax({
url: apiL+'/api/wx/address/find',
type: 'get',
timeout : 10000,
dataType: 'json',
data: {userId:userId(),type:2,requestTokenId:requestTokenId()},
success:function(data){
if (data.success) {
if (data.info.length) {
selfReceAddr(data);
}else{
noshipsearch();
};
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','selfDeliveryBookA()');
}else if(status=='timeout'){
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','selfDeliveryBookA()');
}
}
});
}else{
alert(data.message)
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
fubottom('保存失败,请重试');
}else if(status=='timeout'){
fubottom('保存失败,请重试');
}
return false;
}
});
}else{
AMap.service('AMap.Geocoder',function(){//回调函数
//实例化Geocoder
var geocoder = new AMap.Geocoder({
// city: "010"//城市,默认:“全国”
});
geocoder.getLocation(aeraArr[0]+""+aeraArr[1]+""+aeraArr[2], function(status, result) {
loadingRemove();
if (status === 'complete' && result.info === 'OK') {
loading('提交中...');
var data={
userId:userId(),
type:2,
id:id,
company:company,
contact:contact,
phone:phone,
proName:aeraArr[0],
proNumber:aeraNumArr[0],
cityName:aeraArr[1],
cityNumber:aeraNumArr[1],
areaName:aeraArr[2],
areaNumber:aeraNumArr[2],
detailed:detailed,
lat:result.geocodes[0].location.lat,
lng:result.geocodes[0].location.lng,
isDefault:0,
requestTokenId:requestTokenId()
}
$.ajax({
url: apiL+'/api/wx/address/edit',
type: 'get',
timeout : 10000,
dataType: 'json',
data: data,
success:function(data){
loadingRemove();
if (data.success) {
$('.self_shou_edit').remove();
$.ajax({
url: apiL+'/api/wx/address/find',
type: 'get',
timeout : 10000,
dataType: 'json',
data: {userId:userId(),type:2,requestTokenId:requestTokenId()},
success:function(data){
if (data.success) {
if (data.info.length) {
selfReceAddr(data);
}else{
noshipsearch();
};
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','selfDeliveryBookA()');
}else if(status=='timeout'){
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','selfDeliveryBookA()');
}
}
});
}else{
alert(data.message)
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
fubottom('保存失败,请重试');
}else if(status=='timeout'){
fubottom('保存失败,请重试');
}
return false;
}
});
}else{
$.MsgBox.Alert("地址获取失败,请重试或修改地址后再试",'确定');
}
});
})
}
});
})
}
}
/*添加收货地址*/
function addshouEdit(){
/*$('.self_shou_book').remove();*/
var html=template('self_shou_edit');
$('body').append(html);
$('.self_help_w').animate({right:0}, 300);
$('[name="addBook"]').attr('checked', 'checked');
$('.selfRedSubmit').attr('onclick', 'selfAddRedSubmit()');
addr_aera2();
}
/*添加收货地址列表提交*/
function selfAddRedSubmit(){
if (!noNullfa($('[name="client"]'),'单位名称')) {
noNullfa($('[name="client"]'),'单位名称');
return false;
}else if(!telNo($('[name="tel"]'))){
telNo($('[name="tel"]'))
return false;
}else if(!noNull($('[name="aeraNum"]'),'所在省、市、区')){
noNull($('[name="aeraNum"]'),'所在省、市、区');
return false;
}else if(!noNullfa($('[name="detail"]'),'详细地址')){
noNullfa($('[name="detail"]'),'详细地址');
return false;
}else{
loading('提交中...');
var company=trim($('[name="client"]').val());
var contact=trim($('[name="name"]').val());
var phone=trim($('[name="tel"]').val());
var aera=$('#addr_aera').html();
var aeraNum=$('[name="aeraNum"]').val();
var detailed=trim($('[name="detail"]').val());
var aeraArr=aera.split(' ');
var aeraNumArr=aeraNum.split(',');
AMap.service('AMap.Geocoder',function(){
var geocoder = new AMap.Geocoder({});
geocoder.getLocation(aeraArr[0]+""+aeraArr[1]+""+aeraArr[2]+""+detailed, function(status, result) {
loadingRemove();
if (status === 'complete' && result.info === 'OK') {
loading('提交中...');
var data={
userId:userId(),
type:2,
company:company,
contact:contact,
phone:phone,
proName:aeraArr[0],
proNumber:aeraNumArr[0],
cityName:aeraArr[1],
cityNumber:aeraNumArr[1],
areaName:aeraArr[2],
areaNumber:aeraNumArr[2],
detailed:detailed,
lat:result.geocodes[0].location.lat,
lng:result.geocodes[0].location.lng,
isDefault:0,
requestTokenId:requestTokenId()
};
// console.log(data)
$.ajax({
url: apiL+'/api/wx/address/add',
type: 'post',
timeout : 10000,
dataType: 'json',
data: data,
success:function(data){
loadingRemove();
if (data.success) {
$('.self_shou_edit').remove();
$.ajax({
url: apiL+'/api/wx/address/find',
type: 'get',
timeout : 10000,
dataType: 'json',
data: {userId:userId(),type:2,requestTokenId:requestTokenId()},
success:function(data){
if (data.success) {
if (data.info.length) {
selfReceAddr(data);
}else{
noshipsearch();
};
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','selfDeliveryBookA()');
}else if(status=='timeout'){
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','selfDeliveryBookA()');
}
}
});
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
fubottom('保存失败,请5秒后重试');
}else if(status=='timeout'){
fubottom('保存失败,请5秒后重试');
}
}
});
}else{
AMap.service('AMap.Geocoder',function(){//回调函数
//实例化Geocoder
var geocoder = new AMap.Geocoder({
// city: "010"//城市,默认:“全国”
});
geocoder.getLocation(aeraArr[0]+""+aeraArr[1]+""+aeraArr[2], function(status, result) {
loadingRemove();
if (status === 'complete' && result.info === 'OK') {
loading('提交中...');
var data={
userId:userId(),
type:2,
company:company,
contact:contact,
phone:phone,
proName:aeraArr[0],
proNumber:aeraNumArr[0],
cityName:aeraArr[1],
cityNumber:aeraNumArr[1],
areaName:aeraArr[2],
areaNumber:aeraNumArr[2],
detailed:detailed,
lat:result.geocodes[0].location.lat,
lng:result.geocodes[0].location.lng,
isDefault:0,
requestTokenId:requestTokenId()
};
// console.log(data)
$.ajax({
url: apiL+'/api/wx/address/add',
type: 'post',
timeout : 10000,
dataType: 'json',
data: data,
success:function(data){
loadingRemove();
if (data.success) {
$('.self_shou_edit').remove();
$.ajax({
url: apiL+'/api/wx/address/find',
type: 'get',
timeout : 10000,
dataType: 'json',
data: {userId:userId(),type:2,requestTokenId:requestTokenId()},
success:function(data){
if (data.success) {
if (data.info.length) {
selfReceAddr(data);
}else{
noshipsearch();
};
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','selfDeliveryBookA()');
}else if(status=='timeout'){
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','selfDeliveryBookA()');
}
}
});
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
fubottom('保存失败,请5秒后重试');
}else if(status=='timeout'){
fubottom('保存失败,请5秒后重试');
}
}
});
}else{
$.MsgBox.Alert("地址获取失败,请重试或修改地址后再试",'确定');
}
});
})
}
});
})
$('.selfRedSubmit').attr('disabled', 'disabled').css('background', '#999');
setTimeout(function(){
$('.selfRedSubmit').removeAttr('disabled').css('background', '#52BB03');
}, 5000);
}
}
function senddistinctive(){
var companyName=$('[name="client"]').val();
var phone=$('[name="tel"]').val();
if (companyName==$('[name="senderName"]').val()) {
loadingRemove();
$.MsgBox.Alert("收货方与发货方单位名称不能相同",'确定');
return false;
};
/*if (phone==$('[name="senderMobile"]').val()) {
loadingRemove();
$.MsgBox.Alert("收货方电话不能与发货方电话相同",'确定');
return false;
};*/
return true;
}
function Recdistinctive(){
var companyName=$('[name="client"]').val();
var phone=$('[name="tel"]').val();
if (companyName==$('[name="receiverName"]').val()) {
loadingRemove();
$.MsgBox.Alert("发货方与收货方单位名称不能相同",'确定');
return false;
};
/*if (phone==$('[name="receiverMobile"]').val()) {
loadingRemove();
$.MsgBox.Alert("发货方电话不能与收货方电话相同",'确定');
return false;
};*/
return true;
}
function sendKeysEnt(){
if (event.keyCode==13){
$('.search_input').blur();
selfAddrsearch()
}
}
function recKeysEnt(){
if (event.keyCode==13){
$('.search_input').blur();
selfAddrShouSearch();
}
}
function popping(value){
if (value.length==0) {
$('.shouTelSearchBg,.shouTelSearch').css('display', 'block');
$('.searchTel').focus();
};
}
function shouTelClose(){
$('.searchTel').val('');
$('.shouTelSearchBg,.shouTelSearch').css('display', 'none');
}
// 收货筛选
function shouTelSearch(){
if (telNo($('[name="tel"]'))) {
loading('搜索中...');
var tel=$('[name="tel"]').val();
var base = new Base64();
var result = base.decode(sess.info);
var json=JSON.parse(result);
/*var cityId=json.cityNumber;
var areaId=json.areaNumber;*/
var cityId=$('[name="senderCityId"]').val();
var areaId=$('[name="senderAreaId"]').val();
if (cityId) {
$.ajax({
url: apiAnY+'/api/wx/guest/list/search',
type: 'get',
timeout : 10000,
dataType: 'json',
data: {tel:tel,areaId:areaId,cityId:cityId,requestTokenId:requestTokenId()},
success:function(data){
loadingRemove();
if (data.success) {
var info=data.info;
// console.log(data);
if (info instanceof Array) {
if (info.length==0) {
$.MsgBox.Alert("未检索到收货方信息<br/>您可修改电话重新搜索,或者直接填写收货方信息",'我知道了');
}else{
var html=template('self_shou_list',data);
$('body').append(html);
$('.self_help_w').animate({right:0}, 300);
$('.findNum span').html(info.length);
};
}else{
$.MsgBox.Alert("未检索到收货方信息<br/>您可修改电话重新搜索,或者直接填写收货方信息",'我知道了');
};
}else{
alert(data.message)
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
fubottom('请求超时,请重试');
}else if(status=='timeout'){
fubottom('请求超时,请重试');
}
}
});
}else{
loadingRemove();
$.MsgBox.Alert("请先填写发货方信息!",'我知道了');
};
};
}
function clientele(data){
$('.self_shou_add').remove();
var html=template('self_shou_add');
$('body').append(html);
$('.self_help_w').css('right', '0');
$('.consigner_t [name="client"]').val(data.guestName);
$('.consigner_t [name="name"]').val(data.linkman);
$('.consigner_t [name="tel"]').val(data.tel);
$('.consigner_t #addr_aera').html(data.provinceName+' '+data.cityName+' '+data.areaName).removeClass('c999');
$('.consigner_t [name="aeraNum"]').val(data.provinceId+','+data.cityId+','+data.areaId);
$('.consigner_t [name="detail"]').val(data.address);
$('.consigner_t [name="registerTelLin"]').val(data.registerMobile);
$('.consigner_t [name="registerConLin"]').val(data.guestName);
$('.consigner_t [name="receiverIdLin"]').val(data.receiverId);
$('.self_shou_list').remove();
addr_aera2(data.provinceId,data.cityId,data.areaId);
}
<file_sep>/src/js/ucenter.js
'use strict';
/*信息修改提交验证*/
function editInfoSubmit(){
if (!noNullfa($('[name="company"]'),'单位名称')) {
noNullfa($('[name="company"]'),'单位名称');
return false;
}else if(!noNull($('[name="addrAera"]'),'所在省、市、区')){
noNull($('[name="addrAera"]'),'所在省、市、区');
return false;
}else if(!noNullfa($('[name="detail"]'),'详细地址')){
noNullfa($('[name="detail"]'),'详细地址');
return false;
}else{
var company=trim($('[name="company"]').val());
var name=trim($('[name="name"]').val());
var addrAera=$('[name="addrAera"]').val();
var addrAeraNum=$('[name="addrAeraNum"]').val();
var addrDetail=trim($('[name="detail"]').val());
addrAera=addrAera.split(' ');
addrAeraNum=addrAeraNum.split(',');
var data={
userId:userId(),
company:company,
proName: addrAera[0],
proNumber: addrAeraNum[0],
cityName: addrAera[1],
cityNumber: addrAeraNum[1],
areaName: addrAera[2],
areaNumber: addrAeraNum[2],
detailed: addrDetail,
contact: name,
userName: name,
account:account(),
type:type(),
requestTokenId:requestTokenId()
};
$.ajax({
url: apiL+'/api/wx/login/edituser',
type: 'POST',
dataType: 'json',
data: data,
success:function(data){
if (data.success) {
window.location.href="../ucenter/ucenter.html";
}else{
alert(data.message)
};
}
});
}
}
/*保存常用联系人*/
function comTelSubmit(){
if (!GetQueryString('id')) {
var inp=$('[name="tel"]');
var codeDom=$('[name="code"]');
if (!PhoneNo(inp)) {
PhoneNo(inp)
}else if(!verify(codeDom)){
verify(codeDom)
}else{
loading('保存中...');
var phone=inp.val();
var code=codeDom.val();
$.ajax({
url: apiL+'/api/wx/contacts/add_v1',
type: 'POST',
dataType: 'json',
data: {userId:userId,phone:phone,code:code,number:number(),requestTokenId:requestTokenId()},
success:function(data){
loadingRemove();
if (data.success) {
$.MsgBox.AlertL("添加成功",'确定',function(){
window.location.href="../ucenter/common_use_tel.html"
});
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
fubottom('保存失败,请重试');
}else if(status=='timeout'){
fubottom('保存失败,请重试');
}
}
});
};
}else{
var id=GetQueryString('id');
var inp=$('[name="tel"]');
var codeDom=$('[name="code"]');
if (!PhoneNo(inp)) {
PhoneNo(inp)
}else if(!verify(codeDom)){
verify(codeDom)
}else{
loading('保存中...');
var phone=inp.val();
var code=codeDom.val();
$.ajax({
url: apiL+'/api/wx/contacts/edit_v1',
type: 'POST',
dataType: 'json',
data: {userId:userId,phone:phone,code:code,id:id,number:number(),requestTokenId:requestTokenId()},
success:function(data){
loadingRemove();
if (data.success) {
$.MsgBox.AlertL("修改成功",'确定',function(){
window.location.href="../ucenter/common_use_tel.html"
});
}else{
alert(data.message)
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
fubottom('保存失败,请重试');
}else if(status=='timeout'){
fubottom('保存失败,请重试');
}
}
});
}
};
}
function delPhone(e,id){
console.log('x')
$.MsgBox.ConfirmL("确定要删除吗?",'取消','确定',function(){
loading('删除中...');
$.ajax({
url: apiL+'/api/wx/contacts/delete',
type: 'POST',
dataType: 'json',
data: {id:id,number:number(),requestTokenId:requestTokenId()},
success:function(data){
loadingRemove();
if(data.success) {
e.parents('li').remove();
var liLen=$('#telList').children('li').length;
if(liLen<10 && $('footer').length==0) {
$('body').append('<footer><a class="footer_btn" href="../ucenter/common_tel_edit.html">添加</a></footer>');
};
if(liLen==0) {
noResult($('.telListBox'),400,'您还没有添加常用联系电话');
};
}else{
alert(data.message)
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
fubottom('删除失败,请重试');
}else if(status=='timeout'){
fubottom('删除失败,请重试');
}
}
});
},function(){
return false;
})
}
/*选择上传图片预览*/
function previewImage(file,imgNum){
var div = document.getElementById('preview'+imgNum);
if (file.files && file.files[0]){
complainAdd();
div.innerHTML ='<img id=imghead'+imgNum+'>';
var img = document.getElementById('imghead'+imgNum+'');
var reader = new FileReader();
reader.onload = function(evt){img.src = evt.target.result;}
reader.readAsDataURL(file.files[0]);
var preview=$('#preview'+imgNum);
var img_close_w=preview.parents('.yanzRight').children('.img_close_w');
if (!img_close_w.html()) {
var close='<span class="img_close" onclick="complainDelDmg($(this))"></span>';
img_close_w.html(close);
}
}else{
var sFilter='filter:progid:DXImageTransform.Microsoft.AlphaImageLoader(sizingMethod=scale,src="';
file.select();
var src = document.selection.createRange().text;
div.innerHTML = '<img id=imghead'+imgNum+'>';
var img = document.getElementById('imghead'+imgNum);
img.filters.item('DXImageTransform.Microsoft.AlphaImageLoader').src = src;
div.innerHTML = "<div id=divhead"+imgNum+sFilter+src+"\"'></div>";
}
}
/*添加图片站位*/
function complainAdd(){
var len=$('.yanzRight').length;
var yanzNum=$('.yanzRight').eq(len-1).attr('data-num');
var flag=1;
for (var i = 0; i < len; i++) {
var img_src=$('.yanzRight').eq(i).find('img').attr('src');
if (img_src) {
flag++;
};
};
if (len<3&&flag==len) {
var k=parseInt(yanzNum)+1;
var html='<div class="yanzRight" data-num="'+k+'"><form class="formUp"><label>'
+'<input class="images" name="file" onchange="previewImage(this,'+(k)+')" type="file"/><input type="hidden" class="imgsrc">'
+'<div class="img_show" id="preview'+k+'">'
+'<img id="imghead'+k+'" style="display:none;"/>'
+'</div></label></form><div class="img_close_w"></div></div>';
$('.complain_img').append(html)
};
}
/*删除图片*/
function complainDelDmg(e){
e.parents('.yanzRight').remove();
var len=$('.yanzRight').length;
var yanzNum=$('.yanzRight').eq(len-1).attr('data-num');
var flag=1;
for (var i = 0; i < len; i++) {
var img_src=$('.yanzRight').eq(i).find('img').attr('src');
if (img_src) {
flag++;
};
};
if (len==2&&flag==3) {
var k=parseInt(yanzNum)+1;
var html='<div class="yanzRight" data-num="'+k+'"><form class="formUp"><label>'
+'<input class="images" name="file" onchange="previewImage(this,'+(k)+')" type="file"/><input type="hidden" class="imgsrc">'
+'<div class="img_show" id="preview'+k+'">'
+'<img id="imghead'+k+'" style="display:none;"/>'
+'</div></label></form><div class="img_close_w"></div></div>';
$('.complain_img').append(html)
};
}
/*反馈信息不能为空*/
function conNoNull(e,msg){
var val=e.val();
if (val.length==0) {
e.parents('.comment_input').next('.msg').html('<span class="red">'+msg+'不能为空</span>');
e.focus();
return false;
}else{
e.parents('.comment_input').next('.msg').html('');
return true;
}
}
/*反馈提交*/
function complainSubmit(){
if (!conNoNull($('[name="type"]'),'问题类型')) {
conNoNull($('[name="type"]'),'问题类型');
return false;
}else if(!conNoNull($('[name="content"]'),'投诉内容')){
conNoNull($('[name="content"]'),'投诉内容');
return false;
}else if(!comPhoneNo($('[name="phone"]'))){
comPhoneNo($('[name="phone"]'));
return false;
}else{
var type=$('[name="type"]').val();
var content=$('[name="content"]').val();
var phone=$('[name="phone"]').val();
var imgArr=[];
for (var i = 0; i < $('.imgsrc').length; i++) {
var img=$('.imgsrc').eq(i).val();
if (img) {
imgArr.push(img);
};
};
var imgsUrl=imgArr.toString()
var data={
userId:userId,
type:type,
content:content,
phone:phone,
image:imgsUrl,
requestTokenId:requestTokenId()
}
$.ajax({
url: apiL+'/api/wx/feedback/add',
type: 'POST',
dataType: 'json',
data: data,
success:function(data){
if (data.success) {
window.location.href="../ucenter/ucenter.html";
}else{
alert(data.message)
};
}
});
}
}
/*运费券弹框弹出*/
function tickedQrOpen(num,amount){
loading('加载中...');
$.ajax({
url: apiZhao+'/api/platform/coupon/getno',
type: 'POST',
dataType: 'json',
data: {userId:userId(),id:num,requestTokenId:requestTokenId()},
success:function(data){
loadingRemove();
if (data.success) {
// console.log(data);
var html='<div class="ticked_wrap">'
+'<div class="ticked">'
+'<div class="ticked_pop_c"><span id="tickedQrClose"></span>'
+'<div class="share_details"><p>运费抵扣券金额:<b class="red">'+amount+'</b>元</p>'
+'<div><div id="qrcode"></div></div><p class="c999">此二维码有效期为20秒,<b class="blue" id="daoTime">20</b>秒后会对此券号重新生成二维码</p>'
+'</div></div></div></div>';
$('body').append(html);
jQuery('#qrcode').qrcode({text:data.info});
daoTime(num);
}else{
alert(data.message);
$('.ticked_wrap').remove();
tickedList();
};
}
});
}
function daoTime(num){
var motime=20;
var timer=setInterval(function(){
motime--;
if (motime<=0) {
$.ajax({
url: apiZhao+'/api/platform/coupon/getno',
type: 'POST',
dataType: 'json',
data: {userId:userId(),id:num,requestTokenId:requestTokenId()},
success:function(data){
if (data.success) {
// console.log(data.info);
$('#qrcode').html('');
jQuery('#qrcode').qrcode({text:data.info});
}else{
alert(data.message);
$('.ticked_wrap').remove();
tickedList();
clearInterval(timer);
};
}
});
motime=20
}
$('#daoTime').html(motime);
}, 1000);
$('#tickedQrClose').click(function(event) {
$('.ticked_wrap').remove();
tickedList();
clearInterval(timer);
});
}
function exitUser(){
if (sess.info) {
var base = new Base64();
var result = base.decode(sess.info);
var json=JSON.parse(result);
var unionid=json.unionid;
$.MsgBox.Confirmt("<h3 style='margin-bottom:0.05rem'>解除绑定</h3>您确定要解除与该微信的绑定吗?",'确定','取消',function(){
return true;
},function(){
loading('解除绑定中...');
$.ajax({
url: apiL+'/api/wx/login/unboundwechat',
type: 'POST',
dataType: 'json',
data: {unionid:unionid,requestTokenId:requestTokenId()},
success:function(data){
if (data.success) {
console.log(data);
sessionStorage.removeItem("info");
sessionStorage.removeItem("code");
$.MsgBox.AlertL("解绑成功",'好的',function(){
window.location.href='https://open.weixin.qq.com/connect/oauth2/authorize?appid='+appid+'&redirect_uri='+redirect_uri+'/ucenter/ucenter.html&response_type=code&scope=snsapi_userinfo&state=123#wechat_redirect'
});
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
$.MsgBox.Alert("<h3>解除失败</h3><br /><p style='text-align: left;'>原因:可能是请求服务器失败或者网络出了小差错<br/>1、您可以再次解绑试一试<br />2、退出公众号重新再进入试一试<br />如仍未解决,;联系客服:4001821200</p>",'我知道了');
}else if(status=='timeout'){
$.MsgBox.Alert("<h3>解除失败</h3><br /><p style='text-align: left;'>原因:可能是请求服务器失败或者网络出了小差错<br/>1、您可以再次解绑试一试<br />2、退出公众号重新再进入试一试<br />如仍未解决,联系客服:4001821200</p>",'我知道了');
}
}
});
});
};
}
function headImgUpdate(){
var headUrl=$('[name="headImgShow"]').val();
if (headUrl) {
$.ajax({
url: apiL+'/api/wx/hand/add',
type: 'POST',
dataType: 'json',
data: {userId:userId(),headUrl:headUrl,requestTokenId:requestTokenId()},
success:function(data){
if (data.success) {
alert('上传成功');
window.location.href='../../ucenter/ucenter.html';
}else{
alert(data.message);
};
}
});
}else{
window.location.href='../../ucenter/ucenter.html';
};
}<file_sep>/src/js/main.js
/*
* @Author: 三点十多分
* @Date: 2017-07-12 15:11:55
* @Last Modified by: szyg
* @Last Modified time: 2018-06-04 14:11:27
*/
var api=apiL=wxApi(),apiAn=wxApiAn(),apiAnY=wxApiAnY(),wxApiHai=wxApiHai(),apiZhao=wxApiZhao(),apiHaiL=apiHaiL(),sess=sessionStorage,appid=appid(),redirect_uri=redirectUri();
$(function() {
wecatLoading();
/*用微信打开*/
var shou=window.location.pathname;
if(!isWeiXin()&&shou!='/order/share.html'){
window.location.href='../login/not_login.html';
}else if(!isWeiXin()&&shou=='/order/share.html'){
$('.header_box').remove();
}
/*除了分享页面,去掉网页右上角菜单*/
if (shou!='/order/share.html'&&shou!='/shop/share.html') {
document.addEventListener('WeixinJSBridgeReady', function onBridgeReady() {
// 通过下面这个API隐藏右上角按钮
WeixinJSBridge.call('hideOptionMenu');
});
};
/*定义ios手机没有返回按钮*/
var u = navigator.userAgent, app = navigator.appVersion;
var isAndroid = u.indexOf('Android') > -1 || u.indexOf('Adr') > -1;
var isIOS = !!u.match(/\(i[^;]+;( U;)? CPU.+Mac OS X/); //ios终端
if (isIOS) {
$('.header_box').remove();
}
$('input[readonly]').on('focus', function() {
$(this).trigger('blur');
});
});
function wecatLoading(){
var code=GetQueryString('code');
if (code) {
if (!sess.code) {
var callbackUrl=window.location.pathname;
sess.loginUrl=callbackUrl;
loading('加载中...');
$.ajax({
url: api+'/api/wx/login/applogin',
type: 'POST',
dataType: 'json',
timeout : 10000,
data: {code:code,requestTokenId:requestTokenId()},
success:function(data){
if (data.success) {
sess.code=code;
var info=data.info;
if (data.info!=null) {
if (info.user=='null') {
var base = new Base64();
var Nickname = base.encode(info.Nickname);
window.location.href="../../login/login_select.html?Headimgurl="+info.Headimgurl+"&Nickname="+Nickname+"&unionid="+info.unionid;
}else{
if (info.user) {
var user=JSON.parse(info.user);
// console.log(user);
if (user.userState==1&&user.accountState==1) {
$.MsgBox.Confirmt("对不起,您的账号异常,暂不能登录,请联系客服:4001-821200",'拨打电话','关闭',function(){
WeixinJSBridge.call('closeWindow');
},function(){
window.location.href='tel:4001821200';
WeixinJSBridge.call('closeWindow');
});
return false;
}else{
if (user.userState==1&&callbackUrl!='/ucenter/ucenter.html') {
$.MsgBox.Confirmt("对不起,您的账号已被封停,封停原因:"+user.userFreezeReason+",如有疑问,请联系客服:4001-821200",'拨打电话','关闭',function(){
WeixinJSBridge.call('closeWindow');
},function(){
window.location.href='tel:4001821200';
WeixinJSBridge.call('closeWindow');
});
return false;
}else{
var base = new Base64();
var result = base.encode(info.user);
sess.info=result;
// return false;
if (window.location.pathname=='/ucenter/ucenter.html') {
loading('loading...');
finduser();
}else if(window.location.pathname=='/order/send_out.html'){
sendLoginAddr();
}else if(window.location.pathname=='/order/self_help_order.html'){
self_load();
}else{
window.location.href=sess.loginUrl;
};
};
};
}else{
$('.previewimg').remove();
document.write('登录失败,请关闭重新登录');
};
};
}else{
window.location.href='../login/not_login.html'
}
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
noResultErr($('body'),400,'请求超时,请返回重新再试','wecatLoading()');
}else if(status=='timeout'){
noResultErr($('body'),400,'请求超时,请返回重新再试','wecatLoading()');
}
}
});
}else{
}
}
}
function fengting(){
var userFreezeReason=$('[name="userFreezeReason"]').val();
$.MsgBox.Confirmt("对不起,您的账号已被封停,封停原因:"+userFreezeReason+",如有疑问,请联系客服:4001-821200",'拨打电话','关闭',function(){
return false;
},function(){
window.location.href='tel:4001821200';
});
}
/*返回退出微信页面开始*/
function pushHistory(){
var state = {
title: "title",
url: "#"
};
window.history.pushState(state, "title", "");
};
function popstate(){
pushHistory();
var bool=false;
setTimeout(function(){
bool=true;
},1000);
window.addEventListener("popstate", function(e) {
if(bool)
{
WeixinJSBridge.call('closeWindow');
}
pushHistory();
}, false);
}
function popstateUc(){
pushHistory();
var bool=false;
setTimeout(function(){
bool=true;
},1000);
window.addEventListener("popstate", function(e) {
if(bool)
{
window.location.href='../../ucenter/account.html'
}
pushHistory();
}, false);
}
function popstateUcenter(){
pushHistory();
var bool=false;
setTimeout(function(){
bool=true;
},1000);
window.addEventListener("popstate", function(e) {
if(bool)
{
window.location.href='../../ucenter/ucenter.html'
}
pushHistory();
}, false);
}
/*返回退出微信页面结束*/
/*测试*/
/*function wxApi(){
// 测试
var wxApi='http://192.168.0.138:8193';
// 刘本地
// var wxApi='http://192.168.0.64:8193';
// 200
// var wxApi='http://192.168.0.138:18193';
return wxApi;
}
function wxApiAn(){
// 测试
// var wxApiAn='http://192.168.0.241:8191';
// var wxApiAn='http://192.168.0.206:9191';
var wxApiAn='http://tms.3zqp.com';
// var wxApiAn='http://192.168.0.61:8191';
// 200
// var wxApiAn='http://192.168.0.138:19191';
return wxApiAn;
}
function wxApiAnY(){
// 本地
var wxApiAnY='http://tms.3zqp.com';
// var wxApiAnY='http://192.168.0.61:8191';
// 138
// var wxApiAn='http://192.168.0.138:9191';
return wxApiAnY;
}
function wxApiHai(){
// var wxApiHai='http://192.168.0.138:9196';
var wxApiHai='http://tmsimg.3zqp.com';
return wxApiHai;
}
function wxApiZhao(){
// var wxApiZhao='http://appapi.3zqp.com';
// 138
var wxApiZhao='http://192.168.0.138:8192';
// 本地
// var wxApiZhao='http://192.168.0.82:8192';
return wxApiZhao;
}
function apiHaiL(){
var apiHaiL='http://acc.3zqp.com';
// 138
// var apiHaiL='http://192.168.0.138:9199';
// 本地
// var apiHaiL='http://192.168.0.206:9199';
return apiHaiL;
}
function imgShowApi(){
// var imgShowApi='https://img3zxg.oss-cn-beijing.aliyuncs.com/';
var imgShowApi='https://3zxgimg.oss-cn-beijing.aliyuncs.com/';
return imgShowApi;
}
function appid(){
var appid='wx9475251f4c74eccd';
return appid;
}
function redirectUri(){
var redirectUri='http://192.168.0.73';
return redirectUri;
}*/
/*线上*/
/*function wxApi(){var wxApi='http://weixinapi.3zqp.com';return wxApi;}
function wxApiAn(){var wxApiAn='http://tms.3zqp.com';return wxApiAn;}
function wxApiAnY(){var wxApiAnY='http://tms.3zqp.com';return wxApiAnY;}
function wxApiHai(){var wxApiHai='http://tmsimg.3zqp.com';return wxApiHai;}
function imgShowApi(){var imgShowApi='https://3zxgimg.oss-cn-beijing.aliyuncs.com/';return imgShowApi;}
function wxApiZhao(){var wxApiZhao='http://appapi.3zqp.com';return wxApiZhao;}
function apiHaiL(){var apiHaiL='http://acc.3zqp.com';return apiHaiL;}
function appid(){var appid='wx6da56d9844be9ca1';return appid;}
function redirectUri(){var redirectUri='http://weixinweb.3zqp.com';return redirectUri;}
*/
/*准生产*/
function wxApi(){var wxApi='http://pweixinapi.3zqp.com';return wxApi;}
function wxApiAn(){var wxApiAn='http://ptms.3zqp.com';return wxApiAn;}
function wxApiAnY(){var wxApiAnY='http://ptms.3zqp.com';return wxApiAnY;}
function wxApiHai(){var wxApiHai='http://ptmsimg.3zqp.com';return wxApiHai;}
function imgShowApi(){var imgShowApi='https://3zxgimg.oss-cn-beijing.aliyuncs.com/';return imgShowApi;}
function wxApiZhao(){var wxApiZhao='http://pappapi.3zqp.com';return wxApiZhao;}
function apiHaiL(){var apiHaiL='http://pacc.3zqp.com';return apiHaiL;}
function appid(){var appid='wx9d37162460f921d8';return appid;}
function redirectUri(){var redirectUri='http://192.168.0.73';return redirectUri;}
/*测试*/
/*function wxApi(){var wxApi='http://tweixinapi.3zqp.com';return wxApi;}
function wxApiAn(){var wxApiAn='http://ttms.3zqp.com';return wxApiAn;}
function wxApiAnY(){var wxApiAnY='http://ttms.3zqp.com';return wxApiAnY;}
function wxApiHai(){var wxApiHai='http://ttmsimg.3zqp.com';return wxApiHai;}
function imgShowApi(){var imgShowApi='https://3zxgimg.oss-cn-beijing.aliyuncs.com/';return imgShowApi;}
function wxApiZhao(){var wxApiZhao='http://tappapi.3zqp.com';return wxApiZhao;}
function apiHaiL(){var apiHaiL='http://tacc.3zqp.com';return apiHaiL;}
function appid(){var appid='wx9d37162460f921d8';return appid;}
function redirectUri(){var redirectUri='http://192.168.0.73';return redirectUri;}*/
/*微信用户*/
function isWeiXin(){
var ua = window.navigator.userAgent.toLowerCase();
if(ua.match(/MicroMessenger/i) == 'micromessenger'){
return true;
}else{
return false;
}
}
function userId(){
if (sess.info) {
var base = new Base64();
var result = base.decode(sess.info);
var json=JSON.parse(result);
var userId=json.id;
return userId;
}
};
function userTel(){
if (sess.info) {
var base = new Base64();
var result = base.decode(sess.info);
var json=JSON.parse(result);
var userTel=json.tel;
return userTel;
}
};
function userName(){
if (sess.info) {
var base = new Base64();
var result = base.decode(sess.info);
var json=JSON.parse(result);
var userName=json.userName;
return userName;
}
};
function number(){
if (sess.info) {
var base = new Base64();
var result = base.decode(sess.info);
var json=JSON.parse(result);
var number=json.number;
return number;
};
};
function account(){
if (sess.info) {
var base = new Base64();
var result = base.decode(sess.info);
var json=JSON.parse(result);
var account=json.account;
return account;
};
};
function type(){
if (sess.info) {
var base = new Base64();
var result = base.decode(sess.info);
var json=JSON.parse(result);
var type=json.type;
return type;
};
};
function requestTokenId(num){
len =num||32;
var $chars = 'ABCDEFGHJKMNPQRSTWXYZabcdefhijkmnprstwxyz2345678';
var maxPos = $chars.length;
var pwd = '';
for (i = 0; i < len; i++) {
pwd += $chars.charAt(Math.floor(Math.random() * maxPos));
}
return 'wx_tms_'+pwd;
}
function requestTokenInput(){
var html='<input name="requestTokenId" type="hidden" value="'+requestTokenId()+'">';
$('form').append(html);
};
/*详情页复制*/
function clipboard(){
var clipboard = new Clipboard('.details_btn');
clipboard.on('success', function(e) {
$('.waybill_wrap').css('display', 'none');
});
clipboard.on('error', function(e) {
alert('失败');
});
}
/*返回*/
function headerGoback(){
history.back(-1);
}
function showRem(msg){
$('.reminder').remove();
$('body').append('<div class="reminder"><p>'+msg+'</p></div>');
var timer=setTimeout(function(){
clearTimeout(timer);
$('.reminder').fadeOut(500);
$('.reminder').remove();
}, 3000);
};
/*获取地址*/
function addr_aera2(pNum,cNum,dNum){
var city_picker = new mui.PopPicker({layer:3});
city_picker.setData(provs);
if (pNum&&cNum&&dNum) {
for (var i = 0; i < provs.length; i++) {
var PIndex=i;
if (provs[i].value==pNum) {
for (var j = 0; j < provs.length; j++) {
var cIndex=j;
if (cNum==provs[i].child[j].value) {
for (var k = 0; k < provs[i].child[j].child.length; k++) {
var dIndex=k;
if (dNum==provs[i].child[j].child[k].value) {
break;
};
};
break;
};
};
break;
}
};
city_picker.pickers[0].setSelectedIndex(PIndex);
city_picker.pickers[1].setSelectedIndex(cIndex);
city_picker.pickers[2].setSelectedIndex(dIndex);
};
$("#addr_aera").on("tap", function(){
$('input').blur();
setTimeout(function(){
city_picker.show(function(items){
$("#addr_value").val((items[0] || {}).value + "," + (items[1] || {}).value + "," + (items[2].value || items[1].value));//该ID为接收城市ID字段
$("#addr_aera").removeClass('c999').html((items[0] || {}).text + " " + (items[1] || {}).text + " " + (items[2].text || items[1].text));
});
},200);
});
}
/*以下是注册页面开始*/
/*获取验证码*/
function sub_tel(e){
if (PhoneNo($('[name="tel"]'))) {
loading('获取中...');
var lonin_tel=$('[name="tel"]').val();
$.ajax({
url: apiL+'/api/wx/validation/getcode',
type: 'POST',
dataType: 'json',
data: {phone:lonin_tel,type:0,requestTokenId:requestTokenId()},
success:function(data){
loadingRemove();
if (data.success) {
e.removeAttr('onclick').html('<span id="dao_time">60</span>秒再次获取');
countDown('#dao_time',60);
}else{
alert(data.message);
};
}
});
};
}
/*验证倒计时*/
function countDown(e,num){
var num=num;
var timer=setInterval(function(){
num--;
$(e).html(num);
if (num==0) {
$('.gain').attr('onclick', 'sub_tel($(this))').html('获取验证码');
clearInterval(timer);
};
}, 1000);
}
/*手机号提交验证*/
function telSubmit(){
if (!PhoneNo($('.tel'))) {
PhoneNo($('.tel'));
return false;
}else if(!verify($('.yzm'))){
verify($('.yzm'));
return false;
}else if(!password($('[name="password"]'))){
password($('[name="password"]'));
return false;
}else if(!password2($('[name="password2"]'))){
password2($('[name="password2"]'));
return false;
}else if($('[name="protocol"]:checked').length<1){
alert('请您先勾选同意《三真车联平台注册协议》');
return false;
}else{
/*防止重复提交*/
$('.footer_btn').attr('disabled', 'disabled');
var timer=setTimeout(function(){
clearTimeout(timer);
$('.footer_btn').removeAttr('disabled');
}, 5000);
loading('提交中...');
var login_tel=$('[name="tel"]').val();
var login_code=$('[name="code"]').val();
var login_psw=$("[name=password]").val();
var unionid=GetQueryString('uid');
var data={
unionid: unionid,
phone: login_tel,
code: login_code,
password: <PASSWORD>,
username:login_tel,
registerType:3,
requestTokenId:requestTokenId(),
};
$.ajax({
url: api+'/api/wx/login/register',
type: 'POST',
dataType: 'json',
data: data,
success:function(data){
loadingRemove();
if (data.success) {
if (data.message) {
alert(data.message)
}else{
console.log(data);
var base = new Base64();
var info = base.encode(JSON.stringify(data.info));
sess.info=info;
var base = new Base64();
var result = base.decode(sess.info);
var json=JSON.parse(result);
console.log(json)
// return false;
window.location.href="../login/login_addr.html?unionid="+unionid;
}
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
$('.footer_btn').removeAttr('disabled');
fubottom('提交失败,请重试');
}else if(status=='timeout'){
$('.footer_btn').removeAttr('disabled');
fubottom('提交失败,请重试');
}
}
});
}
}
function protocolClose(){
$('.protocol_wrap').css('display', 'none');
}
function protocolSelect(){
$('[name="protocol"]').attr('checked', 'checked');
$('.protocol_wrap').css('display', 'none');
}
/*地址详情提交验证*/
function addrSubmit(){
if (!noNullfa($('.company'),'公司名称')) {
noNullfa($('.company'),'公司名称');
return false;
}else if(!nameLen($('[name="name"]').val())){
nameLen($('[name="name"]').val());
return false;
}else if(!noNull($('#addr_value'),'所在地址')){
noNull($('#addr_value'),'所在地址');
return false;
}else if(!noNullfa($('.addr_detail'),'详细地址')){
noNullfa($('.addr_detail'),'详细地址');
return false;
}else{
/*防止重复提交*/
$('.footer_btn').attr('disabled', 'disabled');
var timer=setTimeout(function(){
clearTimeout(timer);
$('.footer_btn').removeAttr('disabled');
}, 5000);
loading('提交中...');
var company=trim($('[name="company"]').val());
var name=trim($('[name="name"]').val());
var addrAera=$('#addr_aera').html();
var addrAeraNum=$('[name="addrAeraNum"]').val();
var addrDetail=trim($('[name="addrDetail"]').val());
addrAera=addrAera.split(' ');
addrAeraNum=addrAeraNum.split(',');
var tel=userName();
AMap.service('AMap.Geocoder',function(){
var geocoder = new AMap.Geocoder({});
geocoder.getLocation(addrAera[0]+""+addrAera[1]+""+addrAera[2]+""+addrDetail, function(status, result) {
loadingRemove();
if (status === 'complete' && result.info === 'OK') {
loading('提交中...');
var data={
id:userId(),
company:company,
proName: addrAera[0],
proNumber: addrAeraNum[0],
cityName: addrAera[1],
cityNumber: addrAeraNum[1],
areaName: addrAera[2],
areaNumber: addrAeraNum[2],
detailed: addrDetail,
lat:result.geocodes[0].location.lat,
lng:result.geocodes[0].location.lng,
contact: name,
tel: userTel(),
type:3,
account:account(),
number:number(),
userName:userName(),
unionid:GetQueryString('unionid'),
requestTokenId:requestTokenId()
};
console.log(data)
$.ajax({
url: apiL+'/api/wx/login/adduser',
type: 'POST',
dataType: 'json',
data: data,
success:function(data){
loadingRemove();
// console.log(data);
if (data.success) {
var info=data.info;
if (info!=null) {
info=JSON.stringify(info);
var base = new Base64();
var result = base.encode(info);
sess.info=result;
};
window.location.href="../login/login_ok.html";
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
$('.footer_btn').removeAttr('disabled');
fubottom('提交失败,请重试');
}else if(status=='timeout'){
$('.footer_btn').removeAttr('disabled');
fubottom('提交失败,请重试');
}
}
});
}else{
AMap.service('AMap.Geocoder',function(){//回调函数
//实例化Geocoder
var geocoder = new AMap.Geocoder({
// city: "010"//城市,默认:“全国”
});
geocoder.getLocation(addrAera[0]+""+addrAera[1]+""+addrAera[2], function(status, result) {
loadingRemove();
if (status === 'complete' && result.info === 'OK') {
loading('提交中...');
var data={
id:userId(),
company:company,
proName: addrAera[0],
proNumber: addrAeraNum[0],
cityName: addrAera[1],
cityNumber: addrAeraNum[1],
areaName: addrAera[2],
areaNumber: addrAeraNum[2],
detailed: addrDetail,
lat:result.geocodes[0].location.lat,
lng:result.geocodes[0].location.lng,
contact: name,
tel: userTel(),
type:3,
account:account(),
number:number(),
userName:userName(),
unionid:GetQueryString('unionid'),
requestTokenId:requestTokenId()
};
$.ajax({
url: apiL+'/api/wx/login/adduser',
type: 'POST',
dataType: 'json',
data: data,
success:function(data){
loadingRemove();
// console.log(data);
if (data.success) {
var info=data.info;
if (info!=null) {
info=JSON.stringify(info);
var base = new Base64();
var result = base.encode(info);
sess.info=result;
};
window.location.href="../login/login_ok.html";
}else{
alert(data.message);
};
}
});
}else{
$.MsgBox.Alert("地址获取失败,请重试或修改地址后再试",'确定');
}
});
})
}
});
})
}
}
/*注册完成页面倒计时跳转*/
function login_ok(e,time){
if (!userId()) {
window.location.href="../login/login_tel.html"
};
var i=parseFloat(time)/1000;
if (i>0) {
setInterval(function(){
i--;
e.html(i);
}, 1000);
}else{
e.html(0);
};
setTimeout(function(){
window.location.href=sess.loginUrl;
}, time)
}
/*绑定登录*/
function loginBindSubmit(){
var callbackUrl=sess.loginUrl;
if (!noNull($('[name="account"]'),'账号')) {
$('.msg').html('<span class="red">账号不能为空</span>')
}else if(!noNull($('[name="password"]'),'密码')){
$('.msg').html('<span class="red">密码不能为空</span>')
}else{
loading('绑定中...');
var login_tel=$('[name="account"]').val();
var login_psw=$('[name="password"]').val();
$.ajax({
url: api+'/api/wx/login/relation',
type: 'POST',
dataType: 'json',
data: {username:login_tel,password:<PASSWORD>,unionid:GetQueryString('uid'),requestTokenId:requestTokenId()},
success:function(data){
loadingRemove();
if (data.success) {
// console.log(data);
if (data.message) {
alert(data.message);
}else{
var user=data.info;
// console.log(user)
if (user.userState==1&&user.accountState==1) {
$.MsgBox.Confirmt("对不起,您的账号异常,暂不能登录,请联系客服:4001-821200",'拨打电话','关闭',function(){
WeixinJSBridge.call('closeWindow');
},function(){
window.location.href='tel:4001821200';
WeixinJSBridge.call('closeWindow');
});
return false;
}else{
if (user.userState==1&&callbackUrl!='/ucenter/ucenter.html') {
var base = new Base64();
var result = base.encode(JSON.stringify(data.info));
sess.info=result;
alert('绑定成功');
$.MsgBox.Confirmt("对不起,您的账号已被封停,封停原因:"+user.userFreezeReason+"。您可以进入<a class='blue' href='/ucenter/ucenter.html'>个人中心</a>。如有疑问,请联系客服:4001-821200",'拨打电话','关闭',function(){
WeixinJSBridge.call('closeWindow');
},function(){
window.location.href='tel:4001821200';
WeixinJSBridge.call('closeWindow');
});
return false;
}else{
var base = new Base64();
var result = base.encode(JSON.stringify(data.info));
sess.info=result;
window.location.href=sess.loginUrl;
};
};
};
}else{
alert(data.message);
};
}
});
};
}
/*验证不能为空*/
function noNull(e,msg){
var val=trim(e.val());
if (val.length==0) {
$('.msg').html('<span class="red">'+msg+'不能为空</span>');
return false;
}else{
$('.msg').html('');
return true;
}
}
/*验证不能为空*/
function noNullfa(e,msg){
var val=trim(e.val());
if (val.length==0) {
$('.msg').html('<span class="red">'+msg+'不能为空</span>');
return false;
}else if(notdot(val)){
$('.msg').html('<span class="red">'+msg+'不能输入非法字符</span>');
return false;
}else{
$('.msg').html('');
return true;
}
}
/*删除左右两端的空格*/
function trim(str){
return str.replace(/ /g,'');
}
function nameLen(value){
if (value) {
if (!hanEng(value)) {
$('.msg').html('<span class="red">联系人姓名只能中英文</span>');
return false;
}else{
if (value.length<2||value.length>20) {
$('.msg').html('<span class="red">联系人需输入2-20个字</span>');
return false;
}else{
$('.msg').html('');
return true;
};
};
}else{
$('.msg').html('');
return true;
};
}
/*验证密码*/
function password(e){
var val=e.val();
var val2=$('[name="password2"]').val();
if (val.length==0) {
$('.msg').html('<span class="red">密码不能为空</span>');
return false;
}else{
if (!ispsw(val)) {
$('.msg').html('<span class="red">请输入6-16位数字和字母的组合密码</span>');
return false;
}else if((val2.length!=0) && (val2!=val)){
$('.msg').html('<span class="red">两次输入的密码不一致</span>');
return false;
}else{
$('.msg').html('');
return true;
};
};
}
/*二次密码*/
function password2(e){
var val=e.val();
var val2=$('[name="password"]').val();
if (val.length==0) {
$('.msg').html('<span class="red">重复密码不能为空</span>');
return false;
}else{
if (!ispsw(val)) {
$('.msg').html('<span class="red">请输入重复6-16位数字和字母的组合密码</span>');
return false;
}else if((val2.length!=0) && (val2!=val)){
$('.msg').html('<span class="red">两次输入的密码不一致</span>');
return false;
}else{
$('.msg').html('');
return true;
};
};
}
/*验证电话*/
function telNo(e){
var val=trim(e.val());
if (!isNumberTel(val)) {
loadingRemove();
$('.msg').html('<span class="red">请输入7-12位的手机号或固话</span>');
return false;
}else{
$('.msg').html('');
return true;
};
}
function telNoFa(e){
var val=trim(e.val());
if (!isNumberTel(val)) {
loadingRemove();
$('.msg_fuInputTel').html('<span class="red">请输入7-12位的手机号或固话</span>');
return false;
}else{
$('.msg_fuInputTel').html('');
return true;
};
}
/*验证手机号*/
function PhoneNo(e){
var val=e.val();
if (val.length==0) {
$('.msg').html('<span class="red">手机号不能为空</span>');
return false;
}else{
if (!isPhoneNo(val)) {
$('.msg').html('<span class="red">请输入正确的手机号</span>');
return false;
}else{
$('.msg').html('');
return true;
};
};
}
/*常用联系人验证手机号*/
function comPhoneNo(e){
var val=trim(e.val());
if (val.length==0) {
e.parents('.comment_input').siblings('.msg').html('<span class="red">手机号不能为空</span>');
return false;
}else{
if (!isPhoneNo(val)) {
e.parents('.comment_input').siblings('.msg').html('<span class="red">请输入正确的手机号</span>');
return false;
}else{
e.parents('.comment_input').siblings('.msg').html('');
return true;
};
};
}
/*验证码*/
function verify(e){
var val=e.val();
if (val.length==0) {
$('.msg').html('<span class="red">验证码不能为空</span>');
return false;
}else{
if (!istelverify(val)) {
$('.msg').html('<span class="red">请输入正确的验证码</span>');
return false;
}else{
$('.msg').html('');
return true;
};
};
}
/*验证手机号*/
function isPhoneNo(phone) {
var pattern = /^1\d{10}$/;
return pattern.test(phone);
}
/*验证验证码*/
function istelverify(verify) {
var pattern = /^\d{4,7}$/;
return pattern.test(verify);
}
function isNumberTel(tel){
var pattern = /^\d{7,12}$/;
return pattern.test(tel);
}
/*判断密码为6-16位*/
/*function ispsw(psw) {
var pattern = /^(?![\d]+$)(?![a-zA-Z]+$)(?![\(\)\(\)\,\,\.\、\-\/\\\~\_\&\*\#]+$)[\da-zA-Z\(\)\(\)\,\,\.\、\-\/\\\~\_\&\*\#]{6,16}$/;
return pattern.test(psw);
}*/
function ispsw(psw) {
var pattern = /^(?=.*[A-Za-z])(?=.*\d)[A-Za-z\d]{6,16}$/;
return pattern.test(psw);
}
/*判断密码为6-16位*/
function hanEng(psw) {
var pattern = /^[\u4E00-\u9FA5A-Za-z]+$/;
return pattern.test(psw);
}
/*判断不能为非法字符*/
function notdot(stry) {
var pattern = new RegExp("[`~!@#$^&*=|{}':;',\\[\\].<>/?!@#¥……&*|{}‘;:”“'。,?]");
return pattern.test(stry);
}
/*以上是注册页面结束*/
/*自助下单发货编辑链接*/
function consignerEdit(id){
if (!GetQueryString('waybill')) {
window.location.href="../ucenter/consigner_edit.html?edit="+id+"&from=addr&order="+GetQueryString('selfHp');
}else{
window.location.href="../ucenter/consigner_edit.html?edit="+id+"&from=addr&waybill=addr&order="+GetQueryString('selfHp');
};
}
/*自助下单收货编辑链接*/
function recrivingEdit(id){
if (!GetQueryString('waybill')) {
window.location.href="../ucenter/receiving_edit.html?edit="+id+"&from=addr&order="+GetQueryString('selfHp');
}else{
window.location.href="../ucenter/receiving_edit.html?edit="+id+"&from=addr&waybill=addr&order="+GetQueryString('selfHp');
};
}
/*获取链接参数*/
function GetQueryString(name)
{
var reg = new RegExp("(^|&)"+ name +"=([^&]*)(&|$)");
var r = window.location.search.substr(1).match(reg);
if(r!=null)return unescape(r[2]); return null;
}
function GetQueryString2(name){
var reg = new RegExp("(^|&)"+ name +"=([^&]*)(&|$)");
var r = window.location.search.substr(1).match(reg);
if(r!=null)return unescape(r[1]); return null;
}
function getRequest(){
var url = window.location.search;
var theRequest = new Object();
if (url.indexOf("?") != -1) {
var str = url.substr(1);
strs = str.split("&");
for(var i = 0; i < strs.length; i ++) {
theRequest[strs[i].split("=")[0]]=decodeURI(strs[i].split("=")[1]);
}
}
return theRequest;
}
function loading(text){
var html='<div class="loadingZhuan"><div class="loadingZ"><img src="../skin/images/zhuan.gif"><p>'+text+'</p></div></div>';
$('body').append(html);
}
function loadingRemove(){
$('.loadingZhuan').remove();
}
/*弹出浮层*/
function displayB(e){
e.css('display', 'flex');
}
function displayN(e){
e.css('display', 'none');
}
/*保留小数点后两位*/
function toDecimal2(x) {
var f = parseFloat(x);
if (isNaN(f)) {
return false;
}
var f = Math.round(x*100)/100;
var s = f.toString();
var rs = s.indexOf('.');
if (rs < 0) {
rs = s.length;
s += '.';
}
while (s.length <= rs + 2) {
s += '0';
}
return s;
}
/*时间转换*/
Date.prototype.format = function(format) {
var date = {
"M+": this.getMonth() + 1,
"d+": this.getDate(),
"h+": this.getHours(),
"m+": this.getMinutes(),
"s+": this.getSeconds(),
"q+": Math.floor((this.getMonth() + 3) / 3),
"S+": this.getMilliseconds()
};
if (/(y+)/i.test(format)) {
format = format.replace(RegExp.$1, (this.getFullYear() + '').substr(4 - RegExp.$1.length));
}
for (var k in date) {
if (new RegExp("(" + k + ")").test(format)) {
format = format.replace(RegExp.$1, RegExp.$1.length == 1
? date[k] : ("00" + date[k]).substr(("" + date[k]).length));
}
}
return format;
};
/*三秒消失提示*/
var timerBottom;
function fubottom(text) {
clearTimeout(timerBottom);
if ($('body .fubottom').length>0) {
$('.fubottom').fadeOut().remove();
var html='<div class="fubottom">'+text+'</div>';
$('body').append(html).fadeIn();
}else{
var html='<div class="fubottom">'+text+'</div>';
$('body').append(html).fadeIn();
};
timerBottom=setTimeout(function(){
$('.fubottom').fadeOut().remove();
}, 2000);
}
function noResult(boxDot,height,text){
boxDot.html('<div class="noResult_flex" style="height:'+height+'px"><div class="noResult_flex"><div class="noResult_c"><span><img src="../skin/images/no_find.png" alt=""></span><p>'+text+'</p></div></div>');
}
function noResultErr(boxDot,height,text,func){
boxDot.html('<div class="noResult_flex" style="height:'+height+'px"><div class="noResult_flex" onclick="'+func+'"><div class="noResult_c"><span><img src="../skin/images/loading_erro.png" alt=""></span><p>'+text+'</p></div></div>');
}
<file_sep>/src/js/record.js
'use strict';
// 发货列表
function sendOutList(page){
loading('加载中...');
var search=GetQueryString('search');
var state=GetQueryString('status');
var startTimeCuo=GetQueryString('startTime');
var endTimeCuo=GetQueryString('endTime');
/*获取当前以及一个月前的时间*/
var dataTime=new Date();
var curYear=dataTime.getFullYear();
var curMonth=addZero(dataTime.getMonth()+1);
var curDays=addZero(dataTime.getDate());
var curTime=curYear+'/'+curMonth+'/'+curDays;
var curSerTime=curYear+'-'+curMonth+'-'+curDays;
/*var prevData=new Date(dataTime-2592000000);
var prevYear=prevData.getFullYear();
var prevMonth=addZero(prevData.getMonth()+1);
var prevDays=addZero(prevData.getDate());
var prevTime=prevYear+'/'+prevMonth+'/'+prevDays;
var prevSerTime=prevYear+'-'+prevMonth+'-'+prevDays;*/
// 获取筛选时间
if (startTimeCuo&&endTimeCuo) {
var startTime = new Date();
startTime.setTime(startTimeCuo);
var startTimeShow=startTime.format('yyyy/MM/dd');
var startTimeChange=startTime.format('yyyy-MM-dd');
var endTime = new Date();
endTime.setTime(endTimeCuo);
var endTimeShow=endTime.format('yyyy/MM/dd');
var endTimeChange=endTime.format('yyyy-MM-dd');
}
var searchhtml="";
if (startTime && endTime) {
searchhtml='<span class="swiper-slide">'+startTimeShow+'-'+endTimeShow+'</span>';
};
/*状态*/
if (state===0||state) {
var stateArr=state.split(',');
var selestStatus="";
var selestStatusArr=[];
for (var i = 0; i < stateArr.length; i++) {
if (stateArr[i]=='0') {
selestStatus="待揽货";
}else if(stateArr[i]==1){
selestStatus="已揽货";
}else if(stateArr[i]==2){
selestStatus="运输中";
}else if(stateArr[i]==3){
selestStatus="配送中";
}else if(stateArr[i]==6){
selestStatus="已签收";
}else if(stateArr[i]==4){
selestStatus="取消发货";
}else if(stateArr[i]==5){
selestStatus="已退货";
}else if(stateArr[i]==7){
selestStatus="已驳回";
};
selestStatusArr.push(selestStatus);
};
for (var i = 0; i < selestStatusArr.length; i++) {
searchhtml+='<span class="swiper-slide">'+selestStatusArr[i]+'</span>';
};
};
$('.swiper-wrapper').html(searchhtml);
if (((startTimeCuo&&endTimeCuo)||state===0||state)) {
if (stateArr) {
for (var i = 0; i < stateArr.length; i++) {
$('.filtrateTop ul [value="'+stateArr[i]+'"]').attr('checked', 'checked');
};
};
$('#date_start').val(startTimeChange);
$('#date_end').val(endTimeChange);
var data={
senderId:number(),
pageSize:15,
currentPage:page,
status:state,
startTime:startTimeCuo,
endTime:endTimeCuo,
requestTokenId:requestTokenId()
};
$.ajax({
url: apiAn+'/api/wx/waybill/selectWaybillForSender',
type: 'POST',
dataType: 'json',
timeout : 10000,
data: data,
success:function(data){
if (data.success) {
loadingRemove();
sess.sendPages=data.info.pages
correspCon(data.info,startTimeChange,endTimeChange,state);
}else{
alert(data.message)
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
sess.nowSendPage=1;
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','sendOutList(1)');
$('.loading').html('');
}else if(status=='timeout'){
sess.nowSendPage=1;
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','sendOutList(1)');
$('.loading').html('');
}
}
});
}else{
$.ajax({
url: apiAn+'/api/wx/waybill/selectWaybillForSender',
type: 'POST',
dataType: 'json',
timeout : 10000,
data: {senderId:number(),pageSize:15,currentPage:page,requestTokenId:requestTokenId()},
success:function(data){
loadingRemove();
if (data.success) {
// console.log(data);
sess.sendPages=data.info.pages
correspCon(data.info);
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
sess.nowSendPage=1;
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','sendOutList(1)');
$('.loading').html('');
}else if(status=='timeout'){
sess.nowSendPage=1;
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','sendOutList(1)');
$('.loading').html('');
}
}
});
};
var swiper = new Swiper('.swiper-container', {
scrollbar: '.swiper-scrollbar',
scrollbarHide: true,
slidesPerView: 'auto',
// centeredSlides: true,
// spaceBetween: 10,
grabCursor: true
});
return true;
}
/*收发货搜索列表*/
function corresponSearch(current){
/*获取当前以及一个月前的时间*/
var dataTime=new Date();
var curYear=dataTime.getFullYear();
var curMonth=addZero(dataTime.getMonth()+1);
var curDays=addZero(dataTime.getDate());
var curTime=curYear+'/'+curMonth+'/'+curDays;
/*var prevData=new Date(dataTime-2592000000);
var prevYear=prevData.getFullYear();
var prevMonth=addZero(prevData.getMonth()+1);
var prevDays=addZero(prevData.getDate());
var prevTime=prevYear+'/'+prevMonth+'/'+prevDays;*/
/*获取选中的状态*/
var statu=$('[name="state"]');
var statuArr=[];
for (var i = 0; i < statu.length; i++) {
if (statu.eq(i).attr('checked')) {
statuArr.push(statu.eq(i).val());
};
};
var status=statuArr.toString();
/*当状态为待揽货时*/
if (status===0) {
var statusJ=true;
};
var startTime=$('#date_start').val();
var endTime=$('#date_end').val();
var startArr=startTime.split('-');
var endArr=endTime.split('-');
var searchhtml="";
/*开始结束时间搓*/
var startTimeT=new Date(startArr[0],startArr[1]-1,startArr[2]);
var EndTimeT=new Date(endArr[0],endArr[1]-1,endArr[2],23,59,59);
var startTimeCuo=startTimeT.getTime();
var endTimecuo=EndTimeT.getTime();
if (!startTimeCuo) {
startTimeCuo="";
};
if (!endTimecuo) {
endTimecuo="";
};
if(!startTimeCuo&&endTimecuo){
alert('请选择开始日期');
return false;
}else if(startTimeCuo&&!endTimecuo){
alert('请选择截止日期');
return false;
}
/*当为搜索的时候*/
if ((startTimeCuo&&endTimecuo)||statusJ||status) {
if ((startTimeCuo&&endTimecuo)&&parseInt(endTimecuo)<parseInt(startTimeCuo)) {
alert('结束日期不可大于开始日期');
$('#date_start').val($('#date_end').val());
}else{
var data={
senderId:number(),
pageSize:15,
currentPage:current,
status:status,
startTime:startTimeCuo,
endTime:endTimecuo
}
window.location.href=window.location.pathname+'?search=1&status='+status+'&startTime='+startTimeCuo+'&endTime='+endTimecuo;
};
}else{
window.location.href=window.location.pathname;
};
}
/*发货列表内容*/
function correspCon(info,startTime,endTime,state){
var html="";
var ahtml="";
if (info.list) {
for(var i = 0; i < info.list.length; i++){
var status=info.list[i].status;
var statusShow="";
if (status=='0') {
statusShow="待揽货";
}else if(status==1){
statusShow="已揽货";
}else if(status==2){
statusShow="运输中";
}else if(status==3){
statusShow="配送中";
}else if(status==6){
statusShow="已签收";
}else if(status==4){
statusShow="取消发货";
}else if(status==5){
statusShow="已退货";
}else if(status==7){
statusShow="已驳回";
};
html+='<div class="record_li" data-id="'+info.list[i].id+'"><table>'
+'<col width="52%"><col width="24%"><col width="17%">'
+'<tr><td colspan="2">揽货网点:'+info.list[i].inputDotName+'</td>'
+'<td class="t_r"><span class="red">'+statusShow+'</span></td>'
+'</tr><tr>'
+'<td>运单编号:'+info.list[i].no+'</td>'
+'<td colspan="2">货物件数:'+info.list[i].goodsQuantity+'</td>'
+'</tr><tr>'
+'<td colspan="2">收 货 方:<b>'+info.list[i].receiverName+'</b></td>'
+'<td><a href="tel:'+info.list[i].receiverMobile+'"><img src="../skin/images/tel.png" alt="电话"></a></td>'
+'</tr></table>'
+'<div class="show_details ov_h">';
if (info.list[i].status==0) {
html+='<a class="f_r f14" href="../order/scan.html?order='+info.list[i].no+'">运单条码</a>'
};
html+='<a class="f_r f14" href="../order/logistics.html?order='+info.list[i].no+'">运单详情</a>'
if (info.list[i].status<4) {
html+='<a class="f_r f14" href="../order/self_help_order.html?waybill='+info.list[i].no+'">修改运单</a>'
};
html+='</div></div>';
};
if (info.currentPage==1) {
$('#record_list').html(html);
}else{
$('#record_list').append(html);
};
}else{
$('.loading').html('');
if (state===0) {
var statusJ=true;
};
if ((startTime&&endTime) || statusJ||state) {
if (!(startTime&&endTime)) {
startTime="";
endTime="";
};
if (state.length<1) {
state="";
};
var statuArr=state.split(',');
var stateA=[];
var statu="";
for (var i = 0; i < statuArr.length; i++) {
if (statuArr[i]=='0') {
statu="待揽货";
}else if(statuArr[i]==1){
statu="已揽货";
}else if(statuArr[i]==2){
statu="运输中";
}else if(statuArr[i]==3){
statu="配送中";
}else if(statuArr[i]==6){
statu="已签收";
}else if(statuArr[i]==4){
statu="取消发货";
}else if(statuArr[i]==5){
statu="已退货";
}else if(statuArr[i]==7){
statu="已驳回";
};
stateA.push(statu);
};
var statusStr=stateA.toString();
$('#record_list').html('<div class="ship_nofind"><span><img src="../skin/images/no_find.png" alt=""></span><p>对不起,没有找到“'+statusStr+''+startTime+' '+endTime+'”的</br>相关信息,请重新输入。</p></div>');
}else{
$('#record_list').html('<div class="ship_nofind"><span><img src="../skin/images/no_find.png" alt=""></span><p>您还没有发货记录</p></div>');
};
}
}
// 收货列表
function recordOutList(page){
loading('加载中...');
var search=GetQueryString('search');
var state=GetQueryString('status');
var startTimeCuo=GetQueryString('startTime');
var endTimeCuo=GetQueryString('endTime');
/*获取当前以及一个月前的时间*/
var dataTime=new Date();
var curYear=dataTime.getFullYear();
var curMonth=addZero(dataTime.getMonth()+1);
var curDays=addZero(dataTime.getDate());
var curTime=curYear+'/'+curMonth+'/'+curDays;
var curSerTime=curYear+'-'+curMonth+'-'+curDays;
/*var prevData=new Date(dataTime-2592000000);
var prevYear=prevData.getFullYear();
var prevMonth=addZero(prevData.getMonth()+1);
var prevDays=addZero(prevData.getDate());
var prevTime=prevYear+'/'+prevMonth+'/'+prevDays;
var prevSerTime=prevYear+'-'+prevMonth+'-'+prevDays;*/
// 获取筛选时间
if (startTimeCuo&&endTimeCuo) {
var startTime = new Date();
startTime.setTime(startTimeCuo);
var startTimeShow=startTime.format('yyyy/MM/dd');
var startTimeChange=startTime.format('yyyy-MM-dd');
var endTime = new Date();
endTime.setTime(endTimeCuo);
var endTimeShow=endTime.format('yyyy/MM/dd');
var endTimeChange=endTime.format('yyyy-MM-dd');
}
/*var startCurTimeCuo=Date.parse(prevData);
var endCurTimeCuo=Date.parse(dataTime);*/
var searchhtml="";
if (startTime && endTime) {
searchhtml+='<span class="swiper-slide">'+startTimeShow+'-'+endTimeShow+'</span>';
}/*else{
searchhtml+='<span class="swiper-slide">'+prevTime+'-'+curTime+'</span>';
};*/
/*状态*/
if (state===0||state) {
var stateArr=state.split(',');
var selestStatus="";
var selestStatusArr=[];
for (var i = 0; i < stateArr.length; i++) {
if (stateArr[i]=='0') {
selestStatus="待揽货";
}else if(stateArr[i]==1){
selestStatus="已揽货";
}else if(stateArr[i]==2){
selestStatus="运输中";
}else if(stateArr[i]==3){
selestStatus="配送中";
}else if(stateArr[i]==6){
selestStatus="已签收";
}else if(stateArr[i]==4){
selestStatus="取消发货";
}else if(stateArr[i]==5){
selestStatus="已退货";
}else if(stateArr[i]==7){
selestStatus="已驳回";
};
selestStatusArr.push(selestStatus);
};
for (var i = 0; i < selestStatusArr.length; i++) {
searchhtml+='<span class="swiper-slide">'+selestStatusArr[i]+'</span>';
};
};
$('.swiper-wrapper').html(searchhtml);
/*$('#date_start').val(prevSerTime);
$('#date_end').val(curSerTime);*/
if (((startTimeCuo&&endTimeCuo)||state=='0'||state)) {
if (stateArr) {
for (var i = 0; i < stateArr.length; i++) {
$('.filtrateTop ul [value="'+stateArr[i]+'"]').attr('checked', 'checked');
};
};
$('#date_start').val(startTimeChange);
$('#date_end').val(endTimeChange);
var data={
pageSize:15,
currentPage:page,
status:state,
startTime:startTimeCuo,
endTime:endTimeCuo,
// receiverMobile:userName(),
receiverId:number(),
requestTokenId:requestTokenId()
};
$.ajax({
url: apiAn+'/api/wx/waybill/list/receiver',
type: 'POST',
timeout : 10000,
dataType: 'json',
data: data,
success:function(data){
if (data.success) {
loadingRemove();
sess.recordPages=data.info.pages;
console.log(data)
receiveCon(data.info,state,startTimeChange,endTimeChange);
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
sess.nowRecPage=1;
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','recordOutList(1)');
$('.loading').html('');
}else if(status=='timeout'){
sess.nowRecPage=1;
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','recordOutList(1)');
$('.loading').html('');
}
}
});
}else{
$.ajax({
url: apiAn+'/api/wx/waybill/list/receiver',
type: 'POST',
timeout : 10000,
dataType: 'json',
data: {
receiverId:number(),
pageSize:15,
currentPage:page,
requestTokenId:requestTokenId()
},
success:function(data){
if (data.success) {
loadingRemove();
console.log(data)
sess.recordPages=data.info.pages;
receiveCon(data.info);
}else{
alert(data.message);
};
},
complete : function(XMLHttpRequest,status){
loadingRemove();
if(status=='error'){
sess.nowRecPage=1;
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','recordOutList(1)');
$('.loading').html('');
}else if(status=='timeout'){
sess.nowRecPage=1;
noResultErr($('.record_list'),400,'请求超时,请点击重新获取','recordOutList(1)');
$('.loading').html('');
}
}
});
};
var swiper = new Swiper('.swiper-container', {
scrollbar: '.swiper-scrollbar',
scrollbarHide: true,
slidesPerView: 'auto',
// spaceBetween: 10,
grabCursor: true
});
return true;
}
/*收货列表内容*/
function receiveCon(info,status,startTime,endTime){
var pages=info.pages;
var html="";
var ahtml="";
if (info.list) {
for(var i = 0; i < info.list.length; i++){
var status=info.list[i].status;
switch(status){
case 0:
status="待揽货"
break;
case 1:
status="已揽货"
break;
case 2:
status="运输中"
break;
case 3:
status="配送中"
break;
case 4:
status="取消发货"
break;
case 5:
status="已退货"
break;
case 6:
status="已签收"
break;
case 7:
status="已驳回"
break;
};
html+='<div class="record_li"><table><col width="52%"><col width="24%"><col width="17%">'
+'<tr><td colspan="2">运单编号:'+info.list[i].no+'</td>'
+'<td class="t_r"><span class="red">'+status+'</span></td></tr>'
+'<tr><td colspan="3">货物件数:'+info.list[i].goodsQuantity+'</td></tr>'
+'<tr><td colspan="2">发 货 方:<b>'+info.list[i].senderName+'</b></td>'
+'<td><a href="tel:'+info.list[i].senderMobile+'"><img src="../skin/images/tel.png" alt="电话"></a></td></tr></table>'
+'<div class="show_details ov_h"><a class="f_r f14" href="../order/logistics2.html?order='+info.list[i].no+'">运单详情</a></div>'
+'</div>';
};
if (info.currentPage==1) {
$('#record_list').html(html);
}else{
$('#record_list').append(html);
};
}else{
$('.loading').html('');
if ((startTime&&endTime) || status) {
if (!(startTime&&endTime)) {
startTime="";
endTime="";
};
if (status.length<1) {
status="";
};
var statuArr=status.split(',');
var state=[];
var statu="";
for (var i = 0; i < statuArr.length; i++) {
if(statuArr[i]=='0'){
statu="未揽货";
}else if(statuArr[i]==1){
statu="已揽货";
}else if(statuArr[i]==2){
statu="运输中";
}else if(statuArr[i]==3){
statu="配送中";
}else if(statuArr[i]==6){
statu="已签收";
}else if(statuArr[i]==4){
statu="取消发货";
}else if(statuArr[i]==5){
statu="已退货";
}else if(statuArr[i]==7){
statu="已驳回";
}else{
statu="";
};
state.push(statu);
};
status=state.toString();
$('#record_list').html('<div class="ship_nofind"><span><img src="../skin/images/no_find.png" alt=""></span><p>对不起,没有找到“'+status+''+startTime+' '+endTime+'”的</br>相关信息,请重新输入。</p></div>');
$('#Pagination').html('');
}else{
$('#record_list').html('<div class="ship_nofind"><span><img src="../skin/images/no_find.png" alt=""></span><p>您还没有收货记录</p></div>');
$('#Pagination').html('');
};
}
}
/*关闭筛选*/
function filetrsHide(){
$('.protocol_pop_send_w').fadeOut(400);
$('.protocol_pop_send').animate({right:'-90%'}, 400);
}
function filetrShow(){
$('.protocol_pop_send_w').fadeIn(400);
$('.protocol_pop_send').animate({right:0}, 400);
}
/*筛选重置*/
function filetrsReset(){
$('[name="state"]').removeAttr('checked');
$('.filetrsTime').val('');
}
/*日期为两位数*/
function addZero(input){
if (input<10) {
return '0'+input;
}else{
return input;
};
}
/*开始时间不能大于当前时间*/
function startTime(value){
// var startTime=$('#date_start').val();
var startArr=value.split('-');
var startTimeT=new Date(startArr[0],startArr[1]-1,startArr[2]);
/*开始结束时间搓*/
var startTimeCuo=startTimeT.getTime();
var dataTime=new Date();
var curYear=dataTime.getFullYear();
var curMonth=addZero(dataTime.getMonth()+1);
var curDays=addZero(dataTime.getDate());
var curTimeT=new Date(curYear,curMonth,curDays);
var curTime=curYear+'-'+curMonth+'-'+curDays;
/*结束时间搓*/
var curTimeCuo=curTimeT.getTime();
if (startTimeCuo>curTimeCuo) {
$('#date_start').val(curTime);
};
}
/*结束日期不能大于当前时间*/
function endTime(value){
// var endTime=$('#date_end').val();
var endArr=value.split('-');
var endTimeT=new Date(endArr[0],endArr[1]-1,endArr[2]);
/*开始结束时间搓*/
var endTimeCuo=endTimeT.getTime();
var dataTime=new Date();
var curYear=dataTime.getFullYear();
var curMonth=addZero(dataTime.getMonth()+1);
var curDays=addZero(dataTime.getDate());
var curTimeT=new Date(curYear,curMonth,curDays);
var curTime=curYear+'-'+curMonth+'-'+curDays;
/*结束时间搓*/
var curTimeCuo=curTimeT.getTime();
if (endTimeCuo>curTimeCuo) {
$('#date_end').val(curTime);
};
} | d9c5491cedc7eecf6b3dfe146fe173eb13891e80 | [
"Markdown",
"JavaScript"
] | 7 | Markdown | sandianshiduofen/Szwechat | a15d8a2fac158cad06bce1baba9d89cba0000f9e | ed5bbcc9a718fb26f4472aba948fdf79268fa81b | |
refs/heads/main | <file_sep># cs33
UCLA CS33 Fall 2019 Nowatzki
<file_sep>/* This function marks the start of the farm */
int start_farm()
{
return 1;
}
void setval_272(unsigned *p)
{
*p = 2462550344U;
}
void setval_192(unsigned *p)
{
*p = 3281293400U;
}
unsigned addval_305(unsigned x)
{
return x + 1481211249U;
}
void setval_378(unsigned *p)
{
*p = 1491912884U;
}
void setval_214(unsigned *p)
{
*p = 3347663020U;
}
unsigned addval_275(unsigned x)
{
return x + 3251079496U;
}
unsigned addval_276(unsigned x)
{
return x + 2428995912U;
}
unsigned addval_365(unsigned x)
{
return x + 3281032280U;
}
/* This function marks the middle of the farm */
int mid_farm()
{
return 1;
}
/* Add two arguments */
long add_xy(long x, long y)
{
return x+y;
}
unsigned addval_169(unsigned x)
{
return x + 2425405833U;
}
unsigned addval_317(unsigned x)
{
return x + 3524837769U;
}
unsigned addval_132(unsigned x)
{
return x + 3526940361U;
}
unsigned getval_273()
{
return 3284830619U;
}
unsigned getval_189()
{
return 3286272328U;
}
unsigned getval_207()
{
return 3269495112U;
}
unsigned getval_149()
{
return 3286272332U;
}
void setval_208(unsigned *p)
{
*p = 3532966537U;
}
unsigned getval_286()
{
return 3221280393U;
}
void setval_201(unsigned *p)
{
*p = 3767093461U;
}
void setval_441(unsigned *p)
{
*p = 3703821961U;
}
unsigned getval_316()
{
return 3229925833U;
}
unsigned addval_311(unsigned x)
{
return x + 3281308297U;
}
unsigned getval_303()
{
return 3527985801U;
}
void setval_185(unsigned *p)
{
*p = 3221804681U;
}
unsigned addval_204(unsigned x)
{
return x + 2464188744U;
}
unsigned getval_113()
{
return 3378566793U;
}
void setval_165(unsigned *p)
{
*p = 3281046153U;
}
unsigned addval_482(unsigned x)
{
return x + 3676357001U;
}
void setval_172(unsigned *p)
{
*p = 3767027861U;
}
unsigned getval_472()
{
return 2462747077U;
}
unsigned getval_467()
{
return 2430634313U;
}
void setval_390(unsigned *p)
{
*p = 3286270280U;
}
unsigned addval_197(unsigned x)
{
return x + 3229139337U;
}
unsigned getval_357()
{
return 2378285449U;
}
unsigned getval_190()
{
return 3680551305U;
}
unsigned getval_401()
{
return 2425476745U;
}
void setval_363(unsigned *p)
{
*p = 3376993929U;
}
void setval_151(unsigned *p)
{
*p = 2425542281U;
}
unsigned addval_106(unsigned x)
{
return x + 3682126473U;
}
unsigned addval_198(unsigned x)
{
return x + 3682910593U;
}
unsigned getval_147()
{
return 2425408169U;
}
/* This function marks the end of the farm */
int end_farm()
{
return 1;
}
| 259812d697926cc5d4ca97b71f46f0a20a4b591c | [
"Markdown",
"C"
] | 2 | Markdown | mvttchan/cs33 | caa798ba0a908f37cbc23cae6d2aa099f7b47164 | b47c83fa801c5b202e6464d255e5d3e198c795ef | |
refs/heads/master | <repo_name>chunkey711/comfort<file_sep>/app/initialize.js
import $ from "jquery"
import swal from 'sweetalert2'
let result = '';
function addValueToString (string, value) {
return value + ', ' + string
}
function removeValueFromString (string, value) {
return string.replace(value + ',', '')
}
$(document).ready(() => {
$('.price-item input[type="checkbox"]').bind('change', function () {
if ($(this).parent().hasClass('price-item--active')) {
$(this).parent().removeClass('price-item--active')
result = removeValueFromString(result, $(this).val())
} else {
$(this).parent().toggleClass('price-item--active')
result = addValueToString(result, $(this).val())
}
$('.sale-input').val(result)
})
$("a.scrollto").click(function() {
var elementClick = $(this).attr("href")
var destination = $(elementClick).offset().top;
jQuery("html:not(:animated),body:not(:animated)").animate({
scrollTop: destination
}, 800);
return false;
});
$('#callback-form-1').submit(function (e) {
e.preventDefault()
$.ajax({
type: "POST",
url: "../mail.php",
data: $(this).serialize()
}).done(function() {
swal({
type: 'success',
title: 'Отлично!',
text: 'Спасибо за заявку, наш менеджер свяжется с Вами в скором времени',
})
})
return false
})
$('#callback-form-2').submit(function (e) {
e.preventDefault()
$.ajax({
type: "POST",
url: "../mail.php",
data: $(this).serialize()
}).done(function () {
swal({
type: 'success',
title: 'Отлично!',
text: 'Спасибо за заявку, наш менеджер свяжется с Вами в скором времени',
})
})
return false
})
$('#callback-form-3').submit(function (e) {
e.preventDefault()
$.ajax({
type: "POST",
url: "../mail.php",
data: $(this).serialize()
}).done(function () {
swal({
type: 'success',
title: 'Отлично!',
text: 'Спасибо за заявку, наш менеджер свяжется с Вами в скором времени',
})
})
return false
})
$('#callback-form-4').submit(function (e) {
e.preventDefault()
$.ajax({
type: "POST",
url: "../mail.php",
data: $(this).serialize()
}).done(function () {
swal({
type: 'success',
title: 'Отлично!',
text: 'Спасибо за заявку, наш менеджер свяжется с Вами в скором времени',
})
})
return false
})
$('#callback-form-5').submit(function (e) {
e.preventDefault()
$.ajax({
type: "POST",
url: "../mail.php",
data: $(this).serialize()
}).done(function () {
swal({
type: 'success',
title: 'Отлично!',
text: 'Спасибо за заявку, наш менеджер свяжется с Вами в блзжайшее время',
})
})
return false
})
$('#callback-form-6').submit(function (e) {
e.preventDefault()
$.ajax({
type: "POST",
url: "../mail.php",
data: $(this).serialize()
}).done(function () {
swal({
type: 'success',
title: 'Отлично!',
text: 'Спасибо за заявку, наш менеджер свяжется с Вами в скором времени',
})
})
return false
})
})
| 14c2a74817bd6eaf505e3d4bb56526eda42fe4d7 | [
"JavaScript"
] | 1 | JavaScript | chunkey711/comfort | feaafd4f1d648b2eebd2c743bad494869f07ff78 | c4ba05ac8e66afa195f60480c02e43bc2ecf1cd3 | |
refs/heads/master | <file_sep>/*
* This file was generated by the Gradle 'init' task.
*
* This generated file contains a sample Java project to get you started.
* For more details take a look at the Java Quickstart chapter in the Gradle
* User Manual available at https://docs.gradle.org/5.3/userguide/tutorial_java_projects.html
*/
buildscript {
dependencies {
classpath group: 'de.dynamicfiles.projects.gradle.plugins', name: 'javafx-gradle-plugin', version: '8.8.2'
}
repositories {
mavenLocal()
mavenCentral()
}
}
plugins {
// Apply the java plugin to add support for Java
id 'java'
// Apply the application plugin to add support for building an application
id 'application'
}
//javafx {
// version = "8.0.0"
// modules = [ 'javafx.controls', 'javafx.fxml']
//}
version = '1.0'
mainClassName = 'automation.FXGUI'
//makes a jar with dependencies
jar {
manifest {
attributes "Main-Class": mainClassName
}
from {
configurations.compile.collect { it.isDirectory() ? it : zipTree(it) }
}
}
sourceSets.main {
java {
srcDir 'src/main/java' //assume that your source codes are inside this path
}
resources {
srcDirs = ['src/main/java', 'src/main/resources']
exclude "**/*.java"
}
}
apply plugin: 'javafx-gradle-plugin'
jfx {
mainClass = mainClassName
vendor = 'AutoRoutine'
}
repositories {
// Use jcenter for resolving your dependencies.
// You can declare any Maven/Ivy/file repository here.
jcenter()
maven {
url "https://raw.github.com/kristian/system-hook/mvn-repo/"
}
mavenLocal()
mavenCentral()
}
dependencies {
// This dependency is found on compile classpath of this component and consumers.
implementation 'com.google.guava:guava:27.0.1-jre'
implementation 'com.1stleg:jnativehook:2.1.0'
implementation 'de.dynamicfiles.projects.gradle.plugins:javafx-gradle-plugin:8.8.2'
// Use JUnit test framework
testImplementation 'junit:junit:4.12'
}<file_sep>package main.java.automation;
import java.awt.AWTException;
import java.awt.Dimension;
import java.awt.Rectangle;
import java.awt.Robot;
import java.awt.Toolkit;
import java.awt.image.BufferedImage;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.util.ArrayList;
import main.java.listener.KeyListener;
import main.java.listener.MouseListener;
import org.jnativehook.GlobalScreen;
import org.jnativehook.NativeHookException;
public class Auto {
// Incomplete Auto class that should be able to automate tasks
// Currently working on implementing Key and Mouse Listeners using jnativehook
private int screenHeight;
private int screenWidth;
private boolean startCapture = false;
private ArrayList<BufferedImage> imgs;
public Auto() {
Dimension screenSize = Toolkit.getDefaultToolkit().getScreenSize();
screenHeight = screenSize.height;
screenWidth = screenSize.width;
}
public void roboAuto(String routinename) throws AWTException, FileNotFoundException {
//This is supposed to open up a txt containing the preprogrammed routines and do it
Robot rb = new Robot();
FileReader file = new FileReader("src/automation/routines.txt");
}
public void learnRoutine() throws AWTException {
//This tracks the mouse and keyboard actions and writes to a txt file
addListeners();
startCapture=true;
while (startCapture==true) {
BufferedImage image = takeScreenshot(new Rectangle(screenWidth, screenHeight));
imgs.add(image);
}
System.out.println("Stopped recording");
try {
GlobalScreen.unregisterNativeHook();
} catch (NativeHookException e) {
e.printStackTrace();
}
}
private static void addListeners() {
try {
GlobalScreen.registerNativeHook();
} catch (NativeHookException e) {
e.printStackTrace();
}
KeyListener keylistener = new KeyListener();
MouseListener mouselistener = new MouseListener();
GlobalScreen.addNativeKeyListener(keylistener);
GlobalScreen.addNativeMouseListener(mouselistener);
}
private BufferedImage takeScreenshot(Rectangle screenRect) throws AWTException {
Robot rb = new Robot();
BufferedImage img = rb.createScreenCapture(screenRect);
return img;
}
public void stopRecording() {
startCapture=false;
}
}
<file_sep># AutoRoutine
[](https://travis-ci.com/rahulkaura2004/AutoRoutine)
[](https://ci.appveyor.com/project/lithomas1/autoroutine)
Created by <NAME> and <NAME>
Improved by Kento Nishi
## Prerequisites
Java 1.8
## OS Support
- [X] Windows
- [X] Mac
- [X] Linux
## Download
Clone the repository with git.
```
git clone https://github.com/rahulkaura2004/AutoRoutine
```
## Execution
1. Install gradle (find installation instructions [here](https://gradle.org/))
2. Navigate to the repository.
3. Run the following command:
```bash
gradlew run
```
All done! The program should build and execute.
<file_sep>package main.java.automation;
import java.awt.AWTException;
import javafx.fxml.FXML;
import javafx.scene.control.Button;
public class MainController {
@FXML
private Button newRoutine;
@FXML
private Button executeRoutine;
private boolean started = false;
private Auto auto;
@FXML
private void newRoutine() {
if (started==true) {
auto.stopRecording();
}
else {
auto = new Auto();
newRoutine.setText("Stop routine");
started=true;
try {
auto.learnRoutine();
} catch (AWTException e) {
e.printStackTrace();
}
}
}
@FXML
private void findExecRoutine() {
}
}
| 6feb4433f9d7d32b2ec82aedbb0e685bf45e00f5 | [
"Markdown",
"Java",
"Gradle"
] | 4 | Gradle | Rahul-Kaura/AutoRoutine | e144d7d1849c70b2d330008d4d921dec71ee446c | a47fbad910e2acfadaa1f210716ad6b44933c041 | |
refs/heads/master | <file_sep>$(window).on('load', function() {
$('.flickity--slider').each(function() {
var options = {
contain: true,
freeScroll: false,
adaptiveHeight: false,
autoPlay: true
};
if ($(this).hasClass('justify-content-start')) {
options.cellAlign = 'left';
} else if ($(this).hasClass('justify-content-center')) {
options.cellAlign = 'center';
} else if ($(this).hasClass('justify-content-end')) {
options.cellAlign = 'right';
} else {
options.cellAlign = 'left';
}
if ($(this).hasClass('flickity--dots')) {
options.pageDots = true;
} else {
options.pageDots = false;
}
if ($(this).hasClass('flickity--nav')) {
options.prevNextButtons = true;
} else {
options.prevNextButtons = false;
}
if ($(this).hasClass('flickity--loop')) {
options.wrapAround = true;
} else {
options.wrapAround = false;
}
$(this).flickity(options);
});
}); | 66fcd6db3ffbb724fbc73b3aa39c5de20f6b2ebe | [
"JavaScript"
] | 1 | JavaScript | michalbialkowski/kdmc | 3065e60b7ab4f00d37ca0992dbe436bafa23fb16 | b4a4cef0d20e2c1456b624203baa9987c3d760c3 | |
refs/heads/master | <file_sep>var StationNetwork = require('../lib/station-network');
var sn = new StationNetwork();
setTimeout(function() {
var path = sn.shortestPathBetween("0", "70");
console.log(path);
process.exit(0);
}, 5000);<file_sep>var Graph = require('../lib/graph');
var Vertex = require('../lib/vertex');
var graph = new Graph();
setTimeout(function() {
var vertex1 = new Vertex('corinthiansItaquera', {
name: '<NAME>',
location: {
latitude: 23.456,
longitude: 46.1234
}
});
var vertex2 = new Vertex('arthurAlvim', {
name: '<NAME>',
location: {
latitude: 24.456,
longitude: 45.12345
}
});
var vertex3 = new Vertex('patriarca', {
name: 'Patriarca',
location: {
latitude: 26.456,
longitude: 49.1234
}
});
var vertex4 = new Vertex('vilaMatilde', {
name: '<NAME>',
location: {
latitude: 21.456,
longitude: 41.1234
}
});
var vertex5 = new Vertex('penha', {
name: 'Penha',
location: {
latitude: 22.456,
longitude: 42.1234
}
});
var vertex6 = new Vertex('se', {
name: 'Sé',
location: {
latitude: 22.456,
longitude: 42.1234
}
});
var vertex7 = new Vertex('belem', {
name: 'belem',
location: {
latitude: 22.456,
longitude: 42.1234
}
});
var vertex8 = new Vertex('bras', {
name: 'bras',
location: {
latitude: 22.456,
longitude: 42.1234
}
});
var vertex9 = new Vertex('luz', {
name: 'luz',
location: {
latitude: 22.456,
longitude: 42.1234
}
});
var vertex10 = new Vertex('marechal', {
name: 'marechal',
location: {
latitude: 22.456,
longitude: 42.1234
}
});
var vertex11 = new Vertex('anaRosa', {
name: '<NAME>',
location: {
latitude: 22.456,
longitude: 42.1234
}
});
var vertex12 = new Vertex('ipiranga', {
name: 'ipiranga',
location: {
latitude: 22.456,
longitude: 42.1234
}
});
var vertex13 = new Vertex('paraiso', {
name: 'paraiso',
location: {
latitude: 22.456,
longitude: 42.1234
}
});
var vertex14 = new Vertex('sacoma', {
name: 'sacoma',
location: {
latitude: 22.456,
longitude: 42.1234
}
});
var vertex15 = new Vertex('liberdade', {
name: 'liberdade',
location: {
latitude: 22.456,
longitude: 42.1234
}
});
var vertex16 = new Vertex('pedroII', {
name: '<NAME>',
location: {
latitude: 22.456,
longitude: 42.1234
}
});
graph.addEdge(vertex1, vertex2, 1);
graph.addEdge(vertex1, vertex3, 1);
graph.addEdge(vertex1, vertex6, 1);
graph.addEdge(vertex2, vertex3, 1);
graph.addEdge(vertex2, vertex4, 1);
graph.addEdge(vertex3, vertex4, 1);
graph.addEdge(vertex3, vertex5, 1);
graph.addEdge(vertex4, vertex5, 1);
graph.addEdge(vertex4, vertex7, 1);
graph.addEdge(vertex5, vertex8, 1);
graph.addEdge(vertex7, vertex9, 1);
graph.addEdge(vertex7, vertex10, 1);
graph.addEdge(vertex8, vertex9, 1);
graph.addEdge(vertex8, vertex11, 1);
graph.addEdge(vertex8, vertex12, 1);
graph.addEdge(vertex9, vertex10, 1);
graph.addEdge(vertex10, vertex11, 1);
graph.addEdge(vertex10, vertex13, 1);
graph.addEdge(vertex11, vertex12, 1);
graph.addEdge(vertex11, vertex15, 1);
graph.addEdge(vertex11, vertex16, 1);
graph.addEdge(vertex13, vertex14, 1);
graph.addEdge(vertex15, vertex16, 1);
graph.print();
var DepthFirstSearch = require('../lib/dfs');
var dfs = new DepthFirstSearch(graph, vertex1.vertexId);
var present = false;
console.log("Is " + vertex1.vertexId + " in Graph?");
present = dfs.isVertexIdInGraph(vertex1.vertexId);
console.log("Is in Graph: " + present);
console.log("=========================");
console.log();
console.log("Is " + vertex2.vertexId + " in Graph?");
present = dfs.isVertexIdInGraph(vertex2.vertexId);
console.log("Is in Graph: " + present);
console.log("=========================");
console.log();
console.log("Is " + vertex3.vertexId + " in Graph?");
present = dfs.isVertexIdInGraph(vertex3.vertexId);
console.log("Is in Graph: " + present);
console.log("=========================");
console.log();
console.log("Is " + vertex4.vertexId + " in Graph?");
present = dfs.isVertexIdInGraph(vertex4.vertexId);
console.log("Is in Graph: " + present);
console.log("=========================");
console.log();
console.log("Is " + vertex5.vertexId + " in Graph?");
present = dfs.isVertexIdInGraph(vertex5.vertexId);
console.log("Is in Graph: " + present);
console.log("=========================");
console.log();
console.log("Is " + "santos" + " in Graph?");
present = dfs.isVertexIdInGraph('santos');
console.log("Is in Graph: " + present);
console.log("=========================");
console.log();
console.log("Is " + "paulista" + " in Graph?");
present = dfs.isVertexIdInGraph('paulista');
console.log("Is in Graph: " + present);
console.log("=========================");
console.log();
console.log("Is " + "consolacao" + " in Graph?");
present = dfs.isVertexIdInGraph('consolacao');
console.log("Is in Graph: " + present);
console.log("=========================");
console.log();
console.log("Is " + vertex6.vertexId + " in Graph?");
present = dfs.isVertexIdInGraph(vertex6.vertexId);
console.log("Is in Graph: " + present);
console.log("=========================");
console.log();
console.log("Is " + vertex7.vertexId + " in Graph?");
present = dfs.isVertexIdInGraph(vertex7.vertexId);
console.log("Is in Graph: " + present);
console.log("=========================");
console.log();
console.log("Is " + vertex8.vertexId + " in Graph?");
present = dfs.isVertexIdInGraph(vertex8.vertexId);
console.log("Is in Graph: " + present);
console.log("=========================");
console.log();
console.log("Is " + vertex9.vertexId + " in Graph?");
present = dfs.isVertexIdInGraph(vertex9.vertexId);
console.log("Is in Graph: " + present);
console.log("=========================");
console.log();
console.log("Is " + vertex10.vertexId + " in Graph?");
present = dfs.isVertexIdInGraph(vertex10.vertexId);
console.log("Is in Graph: " + present);
console.log("=========================");
console.log();
console.log("Is " + vertex11.vertexId + " in Graph?");
present = dfs.isVertexIdInGraph(vertex11.vertexId);
console.log("Is in Graph: " + present);
console.log("=========================");
console.log();
console.log("Is " + vertex12.vertexId + " in Graph?");
present = dfs.isVertexIdInGraph(vertex12.vertexId);
console.log("Is in Graph: " + present);
console.log("=========================");
console.log();
console.log("Is " + vertex13.vertexId + " in Graph?");
present = dfs.isVertexIdInGraph(vertex13.vertexId);
console.log("Is in Graph: " + present);
console.log("=========================");
console.log();
console.log("Is " + vertex14.vertexId + " in Graph?");
present = dfs.isVertexIdInGraph(vertex14.vertexId);
console.log("Is in Graph: " + present);
console.log("=========================");
console.log();
}, 10000)<file_sep>var mongoose = require('mongoose');
var Schema = mongoose.Schema;
module.exports = function TrainSystem(stationNetwork) {
this.stationNetwork = stationNetwork || {};
this.locations = {
red: [
[-23.542323, -46.471429], // <NAME>
[-23.542958, -46.475994],
[-23.542397, -46.479578],
[-23.541846, -46.482153],
[-23.540440, -46.484449], // <NAME>
[-23.536818, -46.486729],
[-23.535441, -46.492930],
[-23.533415, -46.497780],
[-23.530484, -46.502672], // Patriarca
[-23.528531, -46.505203],
[-23.527370, -46.509065],
[-23.527842, -46.512778],
[-23.529022, -46.516382], // Guilhermina-Esperança
[-23.530419, -46.519279],
[-23.531324, -46.522648],
[-23.531816, -46.526854],
[-23.531757, -46.531167], // <NAME>
[-23.531639, -46.533034],
[-23.532209, -46.536596],
[-23.533036, -46.540308],
[-23.533606, -46.544084], // Penha
[-23.534344, -46.547797],
[-23.535446, -46.553247],
[-23.536764, -46.559641],
[-23.537551, -46.563847], // Carrão
[-23.537924, -46.566057],
[-23.538692, -46.569748],
[-23.539360, -46.573031],
[-23.540029, -46.576614], // Tatuapé
[-23.540501, -46.578588],
[-23.541210, -46.582300],
[-23.542469, -46.587150],
[-23.543078, -46.589811], // Belém
[-23.543354, -46.593416],
[-23.544337, -46.598244],
[-23.545242, -46.602707],
[-23.546324, -46.607470], // Bresser-Mooca
[-23.546580, -46.608522],
[-23.547052, -46.610775],
[-23.547603, -46.613028],
[-23.547701, -46.615410], // Brás
[-23.548095, -46.617727],
[-23.548724, -46.620753],
[-23.549373, -46.623757],
[-23.549786, -46.625945], // <NAME>
[-23.549904, -46.627136],
[-23.550376, -46.628767],
[-23.550907, -46.631642],
[-23.550632, -46.633284], // Sé
[-23.550150, -46.634990],
[-23.549570, -46.636599],
[-23.548803, -46.638230],
[-23.547927, -46.639324], // Anhangabaú
[-23.547367, -46.640461],
[-23.546531, -46.641534],
[-23.545901, -46.642371],
[-23.544347, -46.642854], // República
[-23.543373, -46.644431],
[-23.542252, -46.646223],
[-23.540856, -46.647897],
[-23.539646, -46.648927], // Santa Cecília
[-23.538210, -46.650665],
[-23.536636, -46.652617],
[-23.534983, -46.654398],
[-23.533764, -46.655986], // <NAME>
[-23.532052, -46.658518],
[-23.529691, -46.661200],
[-23.527704, -46.663518],
[-23.525756, -46.665792] // <NAME>
],
yellow: [
[-23.538135, -46.634334], // Luz
[-23.539827, -46.636480],
[-23.541165, -46.638154],
[-23.543093, -46.640729],
[-23.544347, -46.642854], // República
[-23.547106, -46.646823],
[-23.549820, -46.653131],
[-23.552574, -46.658496],
[-23.555016, -46.662197], // Paulista
[-23.557927, -46.668248],
[-23.561978, -46.674771],
[-23.564575, -46.680050],
[-23.566070, -46.684427], // <NAME>
[-23.566502, -46.685929],
[-23.566896, -46.687431],
[-23.567525, -46.689663],
[-23.567564, -46.692753], // <NAME>
[-23.567289, -46.695457],
[-23.567171, -46.697817],
[-23.566896, -46.700134],
[-23.566512, -46.703090], // Pinheiros
[-23.567495, -46.704506],
[-23.568676, -46.705965],
[-23.570092, -46.707253],
[-23.571508, -46.709012] // Butantã
]
}
/**
* Gets the coordinate of the next location the train is
* to move to.
*/
this.getNextLocationForTrain = function(train) {
var indexOfLocation = this.locationIndexForTrain(train);
/*
* Now we need to take care regarding the following:
*
* 1) If the train is at neither the first location
* nor the last, we can just learn its direction and
* return indexOfLocation + 1 or indexOfLocation - 1.
*
* 2) If the train is at the first position and:
* a) is heading forward, we'll retrieve the
* location at the second position.
*
* b) is moving backwards, we'll also retrieve
* the location at the second position, BUT we'll
* change the direction to "forward".
*
* 3) If the train is at the last location and:
* a) is moving backwards, we'll just retrieve the
* location before the last one.
*
* b) is moving forward, we'll retrieve the location
* before the last one and we'll change the train's
* direction to "backwards".
*/
// 1)
if (indexOfLocation > 0 &&
indexOfLocation < this.locations[train.line].length - 1) {
var nextLatitude = 0;
var nextLongitude = 0;
if (train.direction === 'forward') {
nextLatitude = this.locations[train.line][indexOfLocation + 1][0];
nextLongitude = this.locations[train.line][indexOfLocation + 1][1];
} else {
nextLatitude = this.locations[train.line][indexOfLocation - 1][0];
nextLongitude = this.locations[train.line][indexOfLocation - 1][1];
}
return {
location: {
latitude: nextLatitude,
longitude: nextLongitude
},
direction: train.direction
};
} else if (indexOfLocation === 0) { // 2)
var nextLatitude = this.locations[train.line][indexOfLocation + 1][0];
var nextLongitude = this.locations[train.line][indexOfLocation + 1][1];
var nextDirection = 'forward';
return {
location: {
latitude: nextLatitude,
longitude: nextLongitude
},
direction: nextDirection
};
} else if (indexOfLocation === this.locations[train.line].length - 1) { // 3)
var nextLatitude = this.locations[train.line][indexOfLocation - 1][0];
var nextLongitude = this.locations[train.line][indexOfLocation - 1][1];
var nextDirection = 'backwards';
return {
location: {
latitude: nextLatitude,
longitude: nextLongitude
},
direction: nextDirection
};
}
throw new Error('The train is at an invalid location');
}
/**
* Gets the closest train to the given "path" (origin and destination)
*/
this.getClosestTrainTo = function(origin, destination, callback) {
// Saving a reference to this instance so we can access it
// from within callbacks
self = this;
this.connection = mongoose.createConnection('mongodb://localhost:27017/appmetro');
this.connection.once('open', function() {
self.Train = self.connection.model('Train', new Schema({
_id: Number,
location: Schema.Types.Mixed
}));
/*
* First, we need to know the direction of the train.
* For instance: if origin is Carrão and Destination is Sé,
* the train should be moving forward. Otherwise, if
* origin is Sé and destination is CarrãoM, the train should
* be moving backwards.
* In order to know the direction, we'll use the Station Network
* class.
*/
trainDirection = self.stationNetwork.getDirectionForClosestTrain(origin, destination);
/*
* Then, we need to determine which line the closest train should be
* on.
*/
lineForClosestTrain = self.stationNetwork.getStartLineForPath(origin, destination);
self.Train
.find({direction: trainDirection, line: lineForClosestTrain})
.exec(function(err, docs) {
/*
* In a while, we'll need to know the origin's location
* and line in order to determine the closest train.
* The station variable below will look like the following:
* {
* location: {
* latitude: -23.345231,
* longitude: -46.123345
* },
* line: 'red'
* }
*/
var station = self.stationNetwork.getStationWithStationId(origin);
var locationIndexForStation = self.locationIndexForStation({
location: {
latitude: station.location.latitude,
longitude: station.location.longitude
},
line: lineForClosestTrain
});
/*
* The algorithm below will perform the following steps:
*
* 1 - It iterate through the docs array, and for each
* train, it will: Get the index (in this.locations[line]) of the train's location
* and will store in another array (that relates the index value
* with the train).
*
* 2 - Then, it'll sort the resulting array of step one.
*
* 3 - After having the array sorted, it'll get the train
* that has an index value which is greater than
* the origin station index value and, aat the same time
* is the lowest index value of the array.
*/
var trains = [];
for (var i = 0; i < docs.length; i++) {
// Apparently, docs[i] is NOT a javascript
// object. In order to turn it into an object
// we need to call the toObject() method.
var train = docs[i].toObject();
var indexOfLocation = self.locationIndexForTrain(train);
trains.push({index: indexOfLocation, train: train});
}
// Now we sort the trains array by the index key
// of each object in it.
trains.sort(function(elem1, elem2) {
return elem1.index - elem2.index;
});
/* In order to find out the closest train, here's
* how the algorithm will work:
*
* 1 - If the origin station is the first one, the closest
* train will be the one that has the lowest index in the
* trains array
*
* 2 - If the origin station is the last one, the closest
* train will be the one that has the highest index in
* the trains array.
*
* 3 - If the origin station is neither the first station
* nor the last one and:
*
* a) the requested train should be going forward,
* the chosen train will be the one with index greater
* than the others but less than the origin's index.
*
* b) the requested train should be going backwards,
* the chosen train will be the one with lowest index
* but greater than the origin's index.
*/
var chosenTrain = {};
if (locationIndexForStation === 0) {
chosenTrain = trains[0].train;
} else if (locationIndexForStation === (self.locations[lineForClosestTrain].length - 1)) {
chosenTrain = trains[trains.length - 1].train;
}
if (trainDirection === 'forward') {
var i = 0;
// The following loop gets the train with highest index but
// an index lower than the origin's index
for (; i < trains.length && (trains[i].index <= locationIndexForStation); i++);
/*
* If i is equal to 0, it means there is no train to is
* moving towards the origin station.
*/
if (i === 0)
chosenTrain = {};
else
chosenTrain = trains[i-1].train;
} else if (trainDirection === 'backwards') {
var i = trains.length - 1;
// The following loop gets the train with lowest index but
// an index greater than the origin's index
for (; i > -1 && (trains[i].index >= locationIndexForStation); i--);
/*
* If i is equal to trains.length - 1, it means there is no train to is
* moving towards the origin station.
*/
if (i === (trains.length - 1))
chosenTrain = {};
else
chosenTrain = trains[i+1].train;
}
self.connection.close();
callback(chosenTrain);
});
});
}
/*
* Calculates the distance between the given train and the given
* station. The distance is in meters.
*/
this.distanceBetweenTrainAndStation = function(train, stationId) {
var station = this.stationNetwork.getStationWithStationId(stationId).toObject();
/*
* Before we move on, we need to find out which line
* we'll use to get the distance from the train and the station.
* Recall that a station can have multiple lines, which means that
* we need to pick one. The easiest way is to take the train's line
* and get the index of this line in the lines property of station.
*/
var lineIndex = station.lines.indexOf(train.line);
var line = station.lines[lineIndex];
/*
* Now that we know the line, we need to remove the property
* lines from station and substitute it for the variable line.
*/
delete station["lines"];
station.line = line;
var trainIndex = this.locationIndexForTrain(train);
var stationIndex = this.locationIndexForStation(station);
var totalDistance = 0;
for (var i = trainIndex; i < stationIndex; i++) {
var lat1 = this.locations[station.line][i][0];
var long1 = this.locations[station.line][i][1];
var lat2 = station.location.latitude;
var long2 = station.location.longitude;
totalDistance += this.distanceInMetersBetweenLocations(lat1, long1, lat2, long2);
}
return totalDistance;
}
this.distanceInMetersBetweenLocations = function(latitude1, longitude1, latitude2, longitude2) {
var lat1 = latitude1 * ((Math.PI)/180);
var long1 = longitude1 * ((Math.PI)/180);
var lat2 = latitude2 * ((Math.PI)/180);
var long2 = longitude2 * ((Math.PI)/180);
var deltaPhi = lat2 - lat1;
var deltaLambda = long2 - long1;
var R = 6371000; // Earth's radius in km
var a = Math.sin(deltaPhi/2.0) * Math.sin(deltaPhi/2) + Math.cos(lat1) * Math.cos(lat2) * Math.sin(deltaLambda/2.0) * Math.sin(deltaLambda/2.0);
var c = 2 * Math.atan2(Math.sqrt(a), Math.sqrt(1-a));
var d = R * c;
return d;
}
/**
* Gets the index of the locations array that matches
* its latitude and logitude with the given train's
* latitude and longitude.
*/
this.locationIndexForTrain = function(train) {
var obj = {
location: {
latitude: train.location.latitude,
longitude: train.location.longitude
},
line: train.line
};
return this.getLocationIndex(obj);
}
this.locationIndexForStation = function(station) {
var obj = {
location: {
latitude: station.location.latitude,
longitude: station.location.longitude
},
line: station.line
};
return this.getLocationIndex(obj);
}
this.getLocationIndex = function(obj) {
var indexOfLocation = -1;
this.locations[obj.line].some(function(element, index, array) {
if ((element[0] == obj.location.latitude) && (element[1] == obj.location.longitude))
indexOfLocation = index;
return ((element[0] == obj.latitude) &&
(element[1] == obj.longitude));
});
return indexOfLocation;
}
} <file_sep>module.exports = function(app) {
var stationNetworkController = app.controllers.stationNetwork;
app.get('/stations', stationNetworkController.getStations);
app.get('/stations/shortest-path', stationNetworkController.shortestPath);
}<file_sep>module.exports = function(app) {
var trainSystemController = app.controllers.trainSystem;
app.get('/trains/closest', trainSystemController.closestTrain);
}<file_sep>var express = require('express');
var app = express();
var server = require('http').createServer(app);
var io = require('socket.io')(server);
var MongoOplog = require('mongo-oplog');
var oplog = MongoOplog('mongodb://localhost:27017/local', 'appmetro.trains').tail();
var TrainSystem = require('../lib/train-system');
global.trainId;
var locationChangeDetector = function createLocationChangeDetectorServer(options) {
var port = options.port || 3812;
var trainSystem = new TrainSystem(global.stationNetwork);
server.listen(port, function() {
console.log('Location change detector server is running on port ' + port);
});
io.on('connection', function(socket) {
console.log('There is a new connection!!!');
socket.on('trackTrain', function(trainId) {
global.trainId = trainId;
console.log('Tracking trainId: ' + global.trainId);
oplog.on('update', function(doc) {
if (doc.o2._id === global.trainId) {
console.log('EMITTING EVENT TO TRAIN ID: ' + trainId);
var latitude = doc.o['$set']['location.latitude'];
var longitude = doc.o['$set']['location.longitude'];
socket.emit('trainLocationUpdate', latitude, longitude);
}
});
});
socket.on('trackDistanceBetweenTrainAndStation', function(train, stationId) {
global.trainId = train._id;
global.stationId = stationId;
console.log('Tracking distance between: ' + global.trainId + ' and ' + global.stationId);
oplog.on('update', function(doc) {
if (doc.o2._id === global.trainId) {
var latitude = doc.o['$set']['location.latitude'];
var longitude = doc.o['$set']['location.longitude'];
var updatedTrain = {
location: {
latitude: latitude,
longitude: longitude
},
line: train.line
}
var distance = trainSystem.distanceBetweenTrainAndStation(updatedTrain, stationId);
socket.emit('trainDistanceUpdate', distance);
}
});
});
});
}
module.exports = locationChangeDetector;<file_sep>var TrainSystem = require('../../lib/train-system');
var trainSystem = new TrainSystem(stationNetwork);
var closestTrain = function(req, res) {
var origin = req.query.origin;
var destination = req.query.destination;
trainSystem.getClosestTrainTo(origin, destination, function(train) {
res.status(200).set('Access-Control-Allow-Origin', 'http://localhost:4800').json(train);
});
}
module.exports = function(app) {
var trainSystemController = {
closestTrain: closestTrain
};
return trainSystemController;
}<file_sep>var path = require('path');
var express = require('express');
var app = express();
app.use(express.static(path.join(__dirname, '../../container')));
var createContainerServer = function(options) {
var port = options.port || 3100;
app.listen(port, function() {
console.log('Container website is running on port ' + port);
});
};
module.exports = createContainerServer;<file_sep>var PriorityQueue = require('./priority-queue');
module.exports = function ShortestPath(graph) {
/**
* Graph in which the shortest path algorithm will be
* applied.
*/
this.graph = graph;
this.findShortestPathBetween = function (source, destination) {
var nodes = new PriorityQueue();
var distances = {};
var previous = {};
var path = [];
var smallest;
var vertexId;
var neighbour;
var alt;
var INFINITY = 1/0;
for (vertexId in this.graph.vertices) {
if (vertexId === source) {
distances[vertexId] = 0;
nodes.enqueue(0, vertexId);
} else {
distances[vertexId] = INFINITY;
nodes.enqueue(INFINITY, vertexId);
}
previous[vertexId] = null;
}
while(!nodes.isEmpty()) {
smallest = nodes.dequeue();
if(smallest === destination) {
path;
while(previous[smallest]) {
path.push(smallest);
smallest = previous[smallest];
}
break;
}
if (!smallest || distances[smallest] === INFINITY) {
continue;
}
var adj = this.graph.getAdjacencyList(smallest);
for (var i = 0; i < adj.length; i++) {
/**
* Neighbour example:
* {vertex: "0", weight: 1}
*/
var neighbour = adj[i];
alt = distances[smallest] + neighbour.weight;
if (alt < distances[neighbour.vertex]) {
distances[neighbour.vertex] = alt;
previous[neighbour.vertex] = smallest;
nodes.enqueue(alt, neighbour.vertex);
}
}
}
return path;
}
}<file_sep>var GraphBuilder = require('../lib/graphbuilder');
var mongoose = require('mongoose');
var Schema = mongoose.Schema;
mongoose.connect('mongodb://localhost/appmetro');
var conn = mongoose.connection;
conn.once('open', function() {
mongoose.model('Station', {
_id: Number,
name: String,
location: Schema.Types.Mixed,
nextStations: [{type: Number, ref: 'Station'}]
});
mongoose.model('Station')
.find({})
.populate('nextStations')
.exec(function(err, docs) {
var graphBuilder = new GraphBuilder(docs, {nextStations: 'Array'});
var graph = graphBuilder.build();
graph.print();
console.log(docs);
});
});<file_sep>var path = require('path');
var express = require('express');
var load = require('express-load');
var app = express();
var server = require('http').createServer(app);
// *************************
// S E R V E R S
// *************************
var createLocationChangeDetectorServer = require('./servers/location-change-detector-server');
var createIonicAppServer = require('./servers/ionic-app-server');
var createContainerServer = require('./servers/container-server');
var startLocationUpdaterScript = require('./servers/location-updater-server');
var StationNetwork = require('./lib/station-network');
global.stationNetwork = new StationNetwork();
/*
* Before we move on to set up our server, we need to make sure that
* our station graph was built. The stationNetwork object will have to be
* accessible throughout the app (so the graph is built just once).
*/
stationNetwork.on('stationGraphWasBuilt', function() {
console.log('Station Graph was built!');
load('models', {cwd: 'app'})
.then('controllers', {cwd: 'app'})
.then('routes', {cwd: 'app'})
.into(app);
// Create the location change detector server
createLocationChangeDetectorServer({
port: 3812
});
// Start train location updater script
startLocationUpdaterScript({
port: 3912
});
server.listen(3700, function() {
console.log('Main server is running on port ' + 3700);
});
});
/**
* Calling this function will start the ionic app server, which
* means we'll be able to access the ionic app without running
* ionic serve.
*/
createIonicAppServer({
port: 4800
});
createContainerServer({
port: 3100
});<file_sep>module.exports = function Vertex(vertexId, vertexContent) {
// ********************
// F I E L D S
// ********************
/**
* String or Number that uniquely identifies
* this vertex in a Graph.
*/
this.vertexId = vertexId;
/**
* Content of the vertex (which could be an object, an array, etc).
*/
this.content = vertexContent;
}<file_sep>var Vertex = require('./vertex');
var Graph = require('./graph');
/**
* This class builds a Graph which is a representation of
* the collection passed.
*/
module.exports = function GraphBuilder(docs, edgeIds) {
this.docs = docs;
this.edgeIds = edgeIds;
this.build = function() {
var graph = new Graph();
for (var i = 0; i < this.docs.length; i++) {
/**
* If the _id field of the document is a number,
* we should transform it into a String.
*/
var vertexId = (!isNaN(this.docs[i]._id))
? (this.docs[i]._id.toString())
: (this.docs[i]._id);
var sourceVertex = new Vertex(vertexId, this.docs[i]);
/**
* The connections between vertices in the resulting
* Graph will be defined by the keys in the this.edgeIds
* array. The array looks like the following:
* {
* key1: 'Array',
* key2: 'Object',
* ...
* }
*
* This means that in the this.docs there is a field
* called "key1" which should be used to connect vertices
* and that this field is an array. Besides, there's a "key2"
* field in the docs which represents an object (one-to-one relationship)
*/
for (edgeId in this.edgeIds) {
if (this.edgeIds[edgeId] === 'Array' || this.edgeIds[edgeId] === 'array') {
for (var j = 0; j < this.docs[i][edgeId].length; j++) {
var vertexId = (!isNaN(this.docs[i][edgeId][j].station._id))
? (this.docs[i][edgeId][j].station._id.toString())
: (this.docs[i][edgeId][j].station._id);
var adjacentVertex = new Vertex(vertexId, this.docs[i][edgeId][j].station);
graph.addEdge(sourceVertex, adjacentVertex, this.docs[i][edgeId][j].weight);
}
} else if (this.edgeIds[edgeId] === 'Object' || this.edgeIds[edgeId] === 'object') {
var vertexId = (!isNaN(this.docs[i][edgeId]._id))
? (this.docs[i][edgeId]._id.toString())
: (this.docs[i][edgeId]._id);
var adjacentVertex = new Vertex(vertexId, this.docs[i][edgeId]);
graph.addEdge(sourceVertex, adjacentVertex, 1);
} else {
throw new Error("The Edge ID " + edgeId + " (" + (typeof edgeId) + ") should be either an Array or an Object");
}
}
}
return graph;
}
}<file_sep>var Vertex = require('../lib/vertex');
var Graph = require('../lib/graph');
var ShortestPath = require('../lib/shortest-path');
var graph = new Graph();
var corinthiansItaquera = new Vertex('corinthiansItaquera', {
name: '<NAME>',
location: {
latitude: 23.456,
longitude: 46.1234
}
});
var se = new Vertex('se', {
name: 'Sé',
location: {
latitude: 22.456,
longitude: 42.1234
}
});
var belem = new Vertex('belem', {
name: 'belem',
location: {
latitude: 22.456,
longitude: 42.1234
}
});
var bras = new Vertex('bras', {
name: 'bras',
location: {
latitude: 22.456,
longitude: 42.1234
}
});
var luz = new Vertex('luz', {
name: 'luz',
location: {
latitude: 22.456,
longitude: 42.1234
}
});
var carrao = new Vertex('carrao', {
name: 'Carrão',
location: {
latitude: 22.456,
longitude: 42.1234
}
});
var tatuape = new Vertex('tatuape', {
name: 'Tatuapé',
location: {
latitude: 22.456,
longitude: 42.1234
}
});
var paraiso = new Vertex('paraiso', {
name: 'Paraiso',
location: {
latitude: 22.456,
longitude: 42.1234
}
});
graph.addEdge(corinthiansItaquera, belem, 15);
graph.addEdge(corinthiansItaquera, se, 20);
graph.addEdge(belem, se, 4);
graph.addEdge(se, bras, 5);
graph.addEdge(bras, luz, 10);
graph.addEdge(bras, carrao, 9);
graph.addEdge(luz, tatuape, 20);
graph.addEdge(carrao, tatuape, 22);
graph.addEdge(tatuape, paraiso, 30);
setTimeout(function() {
var dijkstra = new ShortestPath(graph);
var path = dijkstra.findShortestPathBetween(corinthiansItaquera.vertexId, paraiso.vertexId);
path.push(corinthiansItaquera.vertexId);
path.reverse();
console.log(path);
}, 0);<file_sep>var mongoose = require('mongoose');
var Schema = mongoose.Schema;
var TrainSystem = require('./train-system');
module.exports = function TrainLocationUpdater(delay) {
this.delay = delay || 3000;
this.trainSystem = new TrainSystem();
this.start = function() {
var self = this;
this.connection = mongoose.createConnection('mongodb://localhost:27017/appmetro');
this.connection.once('open', function() {
self.Train = self.connection.model('Train', new Schema({
_id: Number,
location: Schema.Types.Mixed
}));
function updateTrainsLocation() {
self.Train
.find({})
.exec(function(err, docs) {
var bulk = self.Train.collection.initializeUnorderedBulkOp();
for (var i = 0; i < docs.length; i++) {
var train = docs[i].toObject();
/*
* result will be like:
*
* {
* location: {
* latitude: <NEW_LATITUDE>,
* longitude: <NEW_LONGITUDE>
* },
* direction: <NEW_DIRECTION>
* }
*/
var result = self.trainSystem.getNextLocationForTrain(train);
// if (train._id === 23) {
// console.log("Train ID: " + train._id);
// console.log("New Location: (" + result.location.latitude + ", " + result.location.longitude + ")");
// console.log("New Direction: " + result.direction);
// console.log();
// console.log();
// }
var findQuery = {_id: train._id};
var updateQuery = {
$set: {
"location.latitude": result.location.latitude,
"location.longitude": result.location.longitude,
"direction": result.direction
}
};
bulk.find(findQuery).update(updateQuery);
}
bulk.execute(function() {
});
});
}
setInterval(function() {
updateTrainsLocation();
}, self.delay);
});
}
}<file_sep>var TrainLocationUpdater = require('../lib/train-location-updater');
setTimeout(function() {
var trainLocationUpdater = new TrainLocationUpdater();
trainLocationUpdater.start();
}, 15000);<file_sep>var mongoose = require('mongoose');
var Schema = mongoose.Schema;
var trainSchema = new Schema({
_id: Number,
location: Schema.Types.Mixed
});
var Train = mongoose.model('Train', trainSchema);
mongoose.connect('mongodb://localhost:27017/appmetro');
mongoose.connection.once('open', function() {
function updateTrainsLocation() {
Train
.find({})
.exec(function(err, docs) {
var bulk = Train.collection.initializeUnorderedBulkOp();
for (var i = 0; i < docs.length; i++) {
var train = docs[i];
var latitude = train.location.latitude + 1;
var longitude = train.location.longitude + 1;
var findQuery = {_id: train._id};
var updateQuery = {$set: {"location.latitude": latitude, "location.longitude": longitude}};
bulk.find(findQuery).update(updateQuery);
}
bulk.execute(function() {
console.log('Finished!');
});
});
}
setInterval(function() {
updateTrainsLocation();
}, 5000);
});<file_sep>var path = require('path');
var express = require('express');
var app = express();
app.use(express.static(path.join(__dirname, '../../client/www')));
var createIonicAppServer = function(options) {
var port = options.port || 4800;
app.listen(port, function() {
console.log('Ionic app server is running on port ' + port);
});
};
module.exports = createIonicAppServer;<file_sep>var mongoose = require('mongoose');
var Schema = mongoose.Schema;
var GraphBuilder = require('./graphbuilder');
var ShortestPath = require('./shortest-path');
var DepthFirstSearch = require('./dfs');
var EventEmitter = require('events').EventEmitter;
var util = require('util');
var StationNetwork = function() {
/**
* The depth first search algorithm requires
* a source at which to start looking for a vertex.
* Since Sé station is pretty much in the center of
* the station network, we'll use it as source.
*
* NOTE: If Sé's ID ever change in the database,
* plase, change it here as well.
*/
this.sourceId = "1200";
this.FORWARD = 'forward';
this.BACKWARDS = 'backwards';
/**
* This function grabs the station collection from
* our database and build the graph that this collection
* represents.
*/
this.initialize = function() {
// Inside mongoose.connection.once, we lose the reference
// to the StationNetwork instance. Inside once(),
// this refers to the mongoose.connection context.
// Since we need to attach the variable Station to
// a StationNetwork instace, we need to store "this"
// in a variable.
var self = this;
/*
* Database connection for this object.
*/
this.connection = mongoose.createConnection('mongodb://localhost:27017/appmetro');
this.connection.once('open', function() {
var nextStationSchema = new Schema({
station: {type: Number, ref: 'Station'},
weight: Number
});
var stationSchema = new Schema({
_id: Number,
name: String,
location: Schema.Types.Mixed,
nextStations: [nextStationSchema]
});
self.Station = self.connection.model('Station', stationSchema);
self.Station
.find({})
.populate('nextStations.station')
.exec(function(err, docs) {
for (var i = 0; i < docs.length; i++) {
docs[i] = docs[i]._doc;
for (var j = 0; j < docs[i].nextStations.length; j++) {
docs[i].nextStations[j] = docs[i].nextStations[j]._doc
}
}
var graphBuilder = new GraphBuilder(docs, {nextStations: 'Array'});
self.graph = graphBuilder.build();
// self.graph.print();
// Let the others know that the Graph
// has already been built.
self.emit('stationGraphWasBuilt');
});
});
}
this.shortestPathBetween = function(origin, destination) {
var shortestPath = new ShortestPath(this.graph);
var path = shortestPath.findShortestPathBetween(origin, destination);
path.push(origin);
path.reverse();
return path;
}
this.getDirectionForClosestTrain = function(origin, destination) {
if (origin === destination) throw new Error("Origin and destination cannot be the same station!");
/*
* If the origin is the first or the last station on a line
* the train direction is not so obvious.
* So firstly, we'll determine whether or not the origin is
* the first or the last station on a a line.
*
* In order to do that, we'll need the origin's neighbours.
*/
var adj = this.graph.getAdjacencyList(origin);
/*
* The neighbours array is not just the origin's neighbour ID (like, 60, 70 etc).
* It looks like the following:
* [{vertex: "60", weight: 1}, {vertex: "70", weight: 1}]
* So we first need to process this array and assemble a new array
* with only vertex IDs.
*/
var neighbours = [];
for (var i = 0; i < adj.length; i++) {
neighbours[i] = parseInt(adj[i].vertex);
}
/*
* Now, in order to know whether the origin is the first or last station
* this is how the following algorithm will work:
*
* 1 - It'll add the origin to the neighbours array
* 2 - It'll sort the neighbours array
* 3 - It'll find out the index of the origin in the sorted neighbours array.
* 4 - If the origin's index is 0, it means it's the first station. Else,
* if the origin's index has the same value of the array's length - 1,
* it means the origin is the last station. Else, it's in the middle.
*/
neighbours.push(parseInt(origin));
neighbours.sort(function(x, y) {return x - y});
var indexOfOrigin = neighbours.indexOf(parseInt(origin));
/*
* Origin will be the first station if indexOfOrigin is 0.
* Else, if indexOfOrigin is equal to neighbours.length - 1,
* it'll be the last station. Else, it's in the middle.
*
* Now, if origin is the first station, then the train's direction
* should be backwards (because there won't be any train that
* will be located off of the line).
* Else, if the origin is the last station, then the train's direction
* should be forward.
*/
if (indexOfOrigin === 0) {
return this.BACKWARDS;
} else if (indexOfOrigin === (neighbours.length - 1)) {
return this.FORWARD;
}
/*
* The first thing we need to do is to get the
* shortest path between origin and destination.
*/
var path = this.shortestPathBetween(origin, destination);
/*
* The direction is determined by the subtraction between
* the second position of the path and the first position
* of the path.
* If the second position has a greater value than the first
* position, it means the train is going forward. Otherwise,
* the train is going backwards.
*
* NOTE: the "numbers" in the path array are actually strings,
* so we need to convert them to numbers before performing
* the subtraction.
*/
var direction = parseInt(path[1]) - parseInt(path[0]);
return (direction > 0) ? (this.FORWARD) : (this.BACKWARDS);
}
/**
* Gets the location and line of the given station (which is
* passed as a string.
*/
this.getStationWithStationId = function(station) {
var dfs = new DepthFirstSearch(this.graph, this.sourceId);
var vertex = dfs.getVertexWithVertexId(station);
return vertex.content;
}
/**
* Gets the line on which the closest train should be.
*/
this.getStartLineForPath = function(origin, destination) {
/*
* To get the line on which the closest train should be,
* the algorithm will perform the following steps:
*
* 1 - It'll get the shortest path between origin and destination.
* 2 - If origin has more than one line, the chosen line will be
* the one of the first element in the shortest path that belongs
* to just one line. For instance, if we are at Sé and wish to go to
* República, there are two possible paths:
*
* a) Sé, Liberdade, ..., Paraíso, ..., Consolação, República
* b) Sé, Anhangabaú and República.
*
* Evidently, the b) path is the shortest. Sé belongs to the red and blue line
* so we ignore it. Next station we'll look at is Anhangabaú. Anhangabaú belongs
* to only the red line. So we'll return the red line. If Anhangabaú belonged
* to 2 lines, we'll keep looking until we ind a station that belongs to just one
* line.
*/
var path = this.shortestPathBetween(origin, destination);
var line = undefined;
var found = false;
for (var i = 0; (i < path.length) && (!found); i++) {
var station = this.getStationWithStationId(path[i]);
console.log(station);
if (station._doc.lines.length === 1) {
line = station._doc.lines[0];
found = !found;
}
}
if (typeof line === 'undefined') throw new Error('Something went wrong, none of the stations in the path array belonged to any line!');
return line;
}
this.getStations = function(callback) {
var self = this;
function getStationsInDatabase() {
self.Station
.aggregate([
{"$unwind": "$lines"},
{
"$group": {
_id: "$lines",
stations: {
"$addToSet": {
_id: "$_id",
name: "$name",
location: "$location",
nextStations: "$nextStations"
}
}
}
}
])
.exec(function(err, docs) {
// Each doc has an _id that holds the entire object
// of interest. So we'll remove this _id and return
// just the doc wrapped by it.
for (var i = 0; i < docs.length; i++) {
docs[i] = docs[i]._id;
}
callback(err, docs);
});
}
// First we need to make sure we still have a connection
if (this.connection.readyState === 1) {
getStationsInDatabase();
}
}
this.getStationModel = function() {
var stationSchema = new Schema({
_id: Number,
name: String,
location: Schema.Types.Mixed,
nextStations: [{type: Number, ref: 'Station'}]
});
return mongoose.model('Station', stationSchema);
}
this.print = function() {
this.graph.print();
}
this.initialize();
}
util.inherits(StationNetwork, EventEmitter);
module.exports = StationNetwork; | 48a248856994bfe52f6702fd8323de028989b4b4 | [
"JavaScript"
] | 19 | JavaScript | renansdias/app-metro-server | d202b5d9a53a452b9214c8fdfb722e5ba4dd46f7 | 21f694e3009a9d2f8e59a375bfa49a813a48f786 | |
refs/heads/master | <repo_name>witcxc/funnycode<file_sep>/go/crawl/a.go
package main
import (
"fmt"
"strconv"
"sync"
)
func main() {
var wg sync.WaitGroup
for i := 0; i < 50; i++ {
wg.Add(1)
go func(si int) {
defer wg.Done()
t := strconv.Itoa(si)
for i := 0; i < 90; i++ {
t += strconv.Itoa(si)
}
fmt.Printf("%s\n", t)
}(i)
}
wg.Wait()
}
<file_sep>/cppcode/trans_gitlog_time.cpp
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <sys/time.h>
#include <xlocale.h>
#include <string.h>
/*
*
* === FUNCTION ======================================================================
* Name: main
* Description:
* =====================================================================================
*/
int main(int argc, char *argv[]) {
struct tm tm_p;
char buf [101];
memset(buf,0,sizeof(buf));
if ( fgets(buf, 40, stdin) != NULL ) {
if (strcmp(argv[1], "git") == 0)
{
strptime(buf, "%a %b %e %T %Y", &tm_p);
// puts(buf);
// printf("%d %d %d %d %d %d\n",tm_p.tm_year,tm_p.tm_mon,tm_p.tm_mday,tm_p.tm_hour,tm_p.tm_min,tm_p.tm_sec);
strftime (buf,40,"%Y-%m-%d %T",&tm_p);
puts(buf);
} else if(strcmp(argv[1], "fmt") == 0)
{
strptime(buf, "%Y-%m-%d %T", &tm_p);
strftime (buf,40,"%Y%m%d%H%M",&tm_p);
puts(buf);
}
}
return EXIT_SUCCESS;
}
<file_sep>/dot/gen_mixer.sh
dot -Tpng mixer.dot -o mixer.png
dot -Tps mixer.dot -o mixer.ps
<file_sep>/compsys/code.c
/*
* =====================================================================================
*
* Filename: code.c
*
* Description:
*
* Version: 1.0
* Created: 2017/06/11 22时56分45秒
* Revision: none
* Compiler: gcc
*
* Author: <NAME> (happyleaf), <EMAIL>
* Organization:
*
* =====================================================================================
*/
int accum = 0;
int sum(int x, int y)
{
int t = x + y;
accum += t;
return t;
}
<file_sep>/cppcode/url_encode.cpp
/*
* =====================================================================================
*
* Filename: url_encode.cpp
*
* Description:
*
* Version: 1.0
* Created: 2017/02/28 00时13分39秒
* Revision: none
* Compiler: gcc
*
* Author: <NAME> (happyleaf), <EMAIL>
* Organization:
*
* =====================================================================================
*/
#include <iostream>
#include <string>
#include <assert.h>
using namespace std;
unsigned char ToHex(unsigned char x)
{
return x > 9 ? x + 55 : x + 48;
}
unsigned char FromHex(unsigned char x)
{
unsigned char y;
if (x >= 'A' && x <= 'Z') y = x - 'A' + 10;
else if (x >= 'a' && x <= 'z') y = x - 'a' + 10;
else if (x >= '0' && x <= '9') y = x - '0';
else assert(0);
return y;
}
std::string UrlEncode(const std::string& str)
{
std::string strTemp = "";
size_t length = str.length();
for (size_t i = 0; i < length; i++)
{
if (isalnum((unsigned char)str[i]) ||
(str[i] == '-') ||
(str[i] == '_') ||
(str[i] == '.') ||
(str[i] == '~'))
strTemp += str[i];
else if (str[i] == ' ')
strTemp += "+";
else
{
strTemp += '%';
strTemp += ToHex((unsigned char)str[i] >> 4);
strTemp += ToHex((unsigned char)str[i] % 16);
}
}
return strTemp;
}
std::string UrlDecode(const std::string& str)
{
std::string strTemp = "";
size_t length = str.length();
for (size_t i = 0; i < length; i++)
{
if (str[i] == '+') strTemp += ' ';
else if (str[i] == '%')
{
assert(i + 2 < length);
unsigned char high = FromHex((unsigned char)str[++i]);
unsigned char low = FromHex((unsigned char)str[++i]);
strTemp += high*16 + low;
}
else strTemp += str[i];
}
return strTemp;
}
#include <stdio.h>
#include <stdlib.h>
/*
* === FUNCTION ======================================================================
* Name: main
* Description:
* =====================================================================================
*/
int main(int argc, char *argv[]) {
string src = "my=apples&are=green+and+red";
string ret = UrlEncode(src);
cout<<"src:["<<src<<"]"<<endl<<"encode:["<<ret<<"]"<<endl;
string dec = UrlDecode(ret);
cout<<dec<<endl;
cout<<"dec:["<<ret<<"]"<<endl<<"src:["<<dec<<"]"<<endl;
return EXIT_SUCCESS;
} /* ---------- end of function main ---------- */
<file_sep>/go/go_equal.go
package main
import (
"encoding/json"
"fmt"
"reflect"
)
type ss struct {
ID int
}
type s struct {
ID ss
name string
}
type DiskSpec struct {
VolumeName string `json:",omitempty"`
AllowUseHostBootDisk bool `json:",omitempty"`
Size int64 //:61440000000 //单位:字节。磁盘空间大小
Type string `json:",omitempty"` //:"SSD", "SATA"
Iops int `json:",omitempty"` //单位:次/秒。应用单实例的磁盘iops配额, NC的iops能力要大于这个值才能被选中;如果后面有iops隔离,那么这个值就是磁盘iops的quota值
Iobps int64 `json:",omitempty"` //单位:字节。应用单实例的磁盘带宽配额, NC的io吞吐能力要大于这个值才能被选中;如果后面有磁盘io带宽隔离,那么这个值就是磁盘带宽的quota值
Rm string `json:",omitempty` //ro:readonly, rw:read write 含义等同于docker -v /a=/b:rw中冒号后面的部分
Exclusive string `json:",omitempty"` //none:不独占,instance:实例独占;app:同一个appname可以共用,不和其他app共用
Mandate bool `json:",omitempty"` //是否必需,默认为 true, 若非必需调度时可能不会给予分配
Driver string `json:",omitempty"` //磁盘driver 类型, 默认为local,
IncludeVolumeInParam bool `json:",omitempty"` // 是否把docker create api 中的-v 选项指定的非bind mount volume 也使用这个磁盘
IncludeVolumeInImage bool `json:",omitempty"` // 是否把镜像中的 volume 也使用这个磁盘
Opt map[string]string `json:",omitempty"` //driver 的选项
Label map[string]string `json:",omitempty"` //磁盘的 label
}
//{ true 1073741824 0 0 true false false map[] map[]}
func main() {
var p, q s
p.ID.ID = 10
p.name = "hello"
q.ID.ID = 11
q.name = "hello"
fmt.Printf("hello!aaa")
if !reflect.DeepEqual(p, q) {
fmt.Printf("equal %v", p)
}
if !reflect.DeepEqual(nil, q) {
fmt.Printf("---- %v, %v", p, nil)
}
var a, b DiskSpec
a.VolumeName = ""
a.AllowUseHostBootDisk = true
a.Size = 1073741824
a.Iops = 0
a.Iobps = 0
a.Mandate = true
a.IncludeVolumeInParam = false
a.IncludeVolumeInImage = false
jsonStr, err := json.Marshal(a)
if err != nil {
fmt.Printf("\nmarshar error!")
}
if err := json.Unmarshal(jsonStr, &b); err != nil {
fmt.Printf("\nun marshar error!")
}
// b.VolumeName = ""
// b.AllowUseHostBootDisk = true
// b.Size = 1073741824
// b.Iops = 0
// b.Iobps = 0
// b.Mandate = true
// b.IncludeVolumeInParam = false
// b.IncludeVolumeInImage = false
if !reflect.DeepEqual(a, b) {
fmt.Printf("\ndisk not equal [%v] != [%v]", a, b)
} else {
fmt.Printf("\nequal [%v] == [%v]", a, b)
}
var c, d map[string]string
if !reflect.DeepEqual(c, d) {
fmt.Printf("\n-----disk not equal [%v] != [%v]", c, d)
}
}
<file_sep>/c/quick_sort.c
/*
* =====================================================================================
*
* Filename: quick_sort.c
*
* Description:
*
* Version: 1.0
* Created: 2017/03/10 18时43分47秒
* Revision: none
* Compiler: gcc
*
* Author: <NAME> (happyleaf), <EMAIL>
* Organization:
*
* =====================================================================================
*/
#include <stdio.h>
#include <stdlib.h>
/*
* === FUNCTION ======================================================================
* Name: quick_sort
* Description:
* =====================================================================================
*/
void quick_sort(int *arr, int size) {
if (size <= 1) {
return;
}
int mark = arr[0];
int s_i = 0;
for (int i = 1; i <= size - 1; ++i ) {
if (arr[i] <= mark) {
int tmp = arr[s_i + 1];
arr[s_i + 1] = arr[i];
arr[i] = tmp;
s_i = s_i + 1;
}
}
arr[0] = arr[s_i];
arr[s_i] = mark;
if(s_i > 1) {
quick_sort(arr, s_i);
}
if ((size - s_i - 1) > 1) {
quick_sort(arr + s_i + 1, size - s_i - 1);
}
} /* ----- end of function quick_sort ----- */
/*
* === FUNCTION ======================================================================
* Name: main
* Description:
* =====================================================================================
*/
int main(int argc, char *argv[]) {
// int arr[5] = {3, 4 , 2, 5, 1};
// printf("%d %d %d %d %d\n", arr[0], arr[1], arr[2], arr[3], arr[4]);
// quick_sort(arr, sizeof(arr)/sizeof(arr[0]));
// printf("%d %d %d %d %d\n", arr[0], arr[1], arr[2], arr[3], arr[4]);
int size = 0;
int * arr = NULL;
int i = 0;
while(scanf("%d", &size) == 1){
arr = (int *)malloc(sizeof(int) * size);
for (i = 0; i < size; ++i)
{
scanf("%d", &(arr[i]));
}
for (i = 0; i < size; ++i) {
printf("%d ", arr[i]);
}
printf("\n");
quick_sort(arr, size);
for (i = 0; i < size; ++i) {
printf("%d ", arr[i]);
}
printf("\n");
}
return EXIT_SUCCESS;
} /* ---------- end of function main ---------- */
<file_sep>/cppcode/self2.c
#include <stdio.h>
char s[] ="#include <stdio.h>%cchar s [] =%c%s%c;main(){printf(s,10,34,s,34);}";main(){printf(s,10,34,s,34);}
<file_sep>/c/gen_sort_data.c
/*
* =====================================================================================
*
* Filename: gen_sort_data.c
*
* Description:
*
* Version: 1.0
* Created: 2017/03/10 19时24分45秒
* Revision: none
* Compiler: gcc
*
* Author: <NAME> (happyleaf), <EMAIL>
* Organization:
*
* =====================================================================================
*/
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <errno.h>
#include <time.h>
/*
* === FUNCTION ======================================================================
* Name: main
* Description:
* =====================================================================================
*/
int main(int argc, char *argv[]) {
FILE *fp; /* output-file pointer */
char *fp_file_name = "data.txt"; /* output-file name */
srand((int)time(0));
fp = fopen( fp_file_name, "w" );
if ( fp == NULL ) {
fprintf ( stderr, "couldn't open file '%s'; %s\n",
fp_file_name, strerror(errno) );
exit (EXIT_FAILURE);
}
int size = rand()%1000000;
fprintf(fp,"%d", size);
for (int i = 0; i < size; ++i) {
fprintf(fp," %d", rand());
}
if( fclose(fp) == EOF ) { /* close output file */
fprintf ( stderr, "couldn't close file '%s'; %s\n",
fp_file_name, strerror(errno) );
exit (EXIT_FAILURE);
}
return EXIT_SUCCESS;
} /* ---------- end of function main ---------- */
<file_sep>/go/single_val.go
package main
import (
"def"
"fmt"
"test"
)
func main() {
fmt.Printf("\nhello[%d]", def.Con)
def.Con += 2
test.Change()
fmt.Printf("\nhello[%d]", def.Con)
}
<file_sep>/clone/prep.sh
#!/bin/bash
yum install git
ssh-keygen -t rsa -b 4096 -C "<EMAIL>"
useradd -m happyleaf
passwd happyleaf
visudo
# add
#username ALL=(ALL) ALL
git clone <EMAIL>:witcxc/funnycode.git
#yum install go
wget https://dl.google.com/go/go1.10.1.linux-amd64.tar.gz
tar -C /usr/local -xzf go$VERSION.$OS-$ARCH.tar.gz
export GOROOT=/usr/local/go
export PATH=$PATH:$GOROOT/bin
export GOPATH=/home/happyleaf/go
go get github.com/shadowsocks/shadowsocks-go/cmd/shadowsocks-server
make all
nohup ./shadowsocks-server -c config.json > shadow_out.log 2>&1 &
sudo bash -c su
nice -n -20 nohup ./serv.bin -c config.json -d
#python
wget https://www.python.org/ftp/python/2.7.14/Python-2.7.14.tgz
tar xzf Python-2.7.14.tgz
cd Python-2.7.14
./configure --prefix=/home/happyleaf/python --enable-optimizations --enable-unicode
make
make install
#virtual env
curl -O https://pypi.python.org/packages/source/v/virtualenv/virtualenv-X.X.tar.gz
tar xvfz virtualenv-X.X.tar.gz
cd virtualenv-X.X
[sudo] python setup.py install
#vim
sudo yum install ncurses-devel
sudo yum install -y ruby ruby-devel lua lua-devel luajit \
luajit-devel ctags git python python-devel \
python3 python3-devel tcl-devel \
perl perl-devel perl-ExtUtils-ParseXS \
perl-ExtUtils-XSpp perl-ExtUtils-CBuilder \
perl-ExtUtils-Embed
git clone https://github.com/vim/vim.git
cd vim/
export PATH=/home/happyleaf/python/bin/:$PATH
make distclean
./configure --with-features=huge \
--enable-multibyte \
--enable-rubyinterp=yes \
--enable-pythoninterp=yes \
--with-python-config-dir=/home/happyleaf/python/lib/python2.7/config \
--enable-perlinterp=yes \
--enable-luainterp=yes \
--enable-cscope \
--prefix=/usr/local
# --enable-python3interp=yes \
# --with-python3-config-dir=/usr/lib/python3.5/config \
#vim Makefile
make
sudo make install
#{
# "server":"0.0.0.0",
# "server_port":8389,
# "local_port":1080,
# "local_address":"127.0.0.1",
# "password":"<PASSWORD>",
# "method": "aes-256-ctr",
# "timeout":600
#}
<file_sep>/dot/gen.sh
dot -Tpng graph.dot -o graph.png
dot -Tpng simple.dot -o simple.png
dot -Tpng module.dot -o module.png
dot -Tpng simple_module.dot -o simple_module.png
dot -Tpng if_sigma.dot -o if_sigma.png
<file_sep>/go/httpserver.go
package main
import (
"bytes"
"fmt"
"io"
"net/http"
)
// hello world, the web server
func HelloServer(w http.ResponseWriter, req *http.Request) {
fmt.Printf("[%v]\n", req)
buf := &bytes.Buffer{}
nRead, err := io.Copy(buf, req.Body)
if err != nil {
fmt.Printf("\nerr[%v]\n", err)
}
fmt.Printf("\nbody[%v]\nlen[%v]\n", buf, nRead)
io.WriteString(w, "hello, world!\n")
}
func main() {
http.HandleFunc("/", HelloServer)
err := http.ListenAndServe(":8089", nil)
if err != nil {
fmt.Printf("\nListenAndServe: %v\n", err)
}
}
<file_sep>/go/compare.go
package main
import (
"fmt"
)
type Person struct {
age int
name string
}
func (Person p) change() int {
p.age += 1
fmt.Printf("\nin func %v", p)
return 0
}
func change(t map[string]int) {
t["hello"] = 13
fmt.Printf("\nin func %v", t)
}
func main() {
var p = Person{12, "hello"}
p.change()
fmt.Printf("\nout %v", p)
a := make(map[string]int)
a["hello"] = 12
change(a)
fmt.Printf("\nout func %v", a)
}
<file_sep>/go/crawl/page_parse.go
package main
import (
"fmt"
"golang.org/x/net/html"
_ "log"
"net/http"
"os"
)
func forEachNode(n *html.Node, pre, post func(n *html.Node)) {
if pre != nil {
pre(n)
}
for c := n.FirstChild; c != nil; c = c.NextSibling {
forEachNode(c, pre, post)
}
if post != nil {
post(n)
}
}
func main() {
resp, err := http.Get(os.Args[1])
if err != nil {
return
}
if resp.StatusCode != http.StatusOK {
resp.Body.Close()
return
}
doc, err := html.Parse(resp.Body)
resp.Body.Close()
if err != nil {
return
}
visitNode := func(n *html.Node) {
if n.Type == html.ElementNode && n.Data == "title" {
fmt.Printf("%+v", n.FirstChild.Data)
}
}
forEachNode(doc, visitNode, nil)
}
| 9b769a18840dbf71f06920fd8b1e0c39c10bc2e9 | [
"C",
"Go",
"C++",
"Shell"
] | 15 | Go | witcxc/funnycode | d9bdc43e1e5c54de0566d88726bf326e7b59daed | a71613e19e11bf33437a5c88fc25e03d885daba4 | |
refs/heads/master | <file_sep>import type { LoaderContext } from "webpack";
export interface PluginContext {
setOutputCSS?: (css: string) => void
}
export interface ExtendedLoaderContext<T = any> extends LoaderContext<T>, PluginContext { }<file_sep>import chai from 'chai'
import sinonChai from 'sinon-chai'
import sinon from 'sinon'
import playwright from 'playwright'
chai.use(sinonChai)
let _browser: playwright.Browser
before(async () => {
_browser = await playwright.chromium.launch();
(global as any)._browser = _browser
})
after(async () => {
await _browser.close()
})
beforeEach(async () => {
sinon.reset()
})<file_sep># Example project React<file_sep>import sinon from 'sinon'
export const loaderContextWithoutOutputCSS = {
resourcePath: '__path__.css',
}
export const loaderContext = {
...loaderContextWithoutOutputCSS,
setOutputCSS: sinon.mock()
}
<file_sep># Writing the Loader
So what are we aiming for? First things first, we want to simply load our CSS from our JS.
Let's create our CSS file and call it `index.css`.
```css
.app {
background: red;
}
```
And of course, use it in the index.js file:
```js
import React from 'react';
import { render } from 'react-dom';
import './index.css'
render(
<div className="app"> Hello World! </div>,
document.getElementById('root')
);
```
Run our application:
```bash
npm run serve
```
Now you probably see this error in the console:\
\
This error makes a lot of sense, as webpack does not know how to handle CSS imports and we need to tell it how.
## Creating Webpack Loader
### What are loaders?
*webpack enables the use of loaders to preprocess files. This allows you to bundle any static resource way beyond JavaScript.*
To put it simply, in our case, they are functions that take the CSS file as input and output a js file.
_**CSS -> JS**_
### Loader implementation
Let's create a file parallel to the `webpack.config.js` named `loader.js`.
Our goal is to append the style value we get from the CSS file inside the dom.
`loader.js`:
```js
// Appending the style inside the head
function appendStyle(value) {
const style = document.createElement('style');
style.textContent = value;
document.head.appendChild(style);
}
// Make sure it is not an arrow function since we will need the `this` context of webpack
function loader(fileValue) {
// We stringify the appendStyle method and creating a file that will invoked with the css file value in run time
return `
(${appendStyle.toString()})(${JSON.stringify(fileValue)})
`
}
module.exports = loader;
```
Now we need to register it inside the webpack config.
`webpack.config.js`:
```js
const config = {
//... rest of the config
module: {
rules: [
// ... other rules not related to CSS
{
test: /\.css$/,
loader: require.resolve('./loader')
}
]
}
// ...
}
```
Restart the terminal, and we got it! 🎊\
\
### What’s happening behind the scenes?
Webpack sees your CSS import inside `index.js`. It looks for a loader and gives it the JavaScript value we want to evaluate in runtime.
## Overcoming the `global` issue
Now we have our style, but everything is global. Every other language solves the global issue with scoping or namespacing.
We will implement the namespace solution. This is going to give us scoping, and each file going to have its own namespace.
For example, our import is going to look like this:
```
AppComponent123__myClass
```
If another component has the same class name, it won't matter behind the scenes since the namespace will be different.
Let's go the `loader.js` and add the following method:
```js
const crypto = require('crypto');
/**
* The name is the class we are going to scope, and the file path is the value we are going to for the namespacing.
*
* The third argument is the classes, a map that points the old name to the new one.
*/
function scope(name, filepath, classes) {
name = name.slice(1); // Remove the dot from the name.
const hash = crypto.createHash('sha1'); // Use sha1 algorithm.
hash.write(filepath); // Hash the filepath.
const namespace = hash.digest('hex').slice(0, 6); // Get the hashed filepath.
const newName = `s${namespace}__${name}`;
classes[name] = newName; // Save the old and the new classes.
return `.${newName}`
}
```
After we are done scoping the class, let's return the loader method.
We need a way to connect the scoped class selector to the user's javascript code.
```js
function loader(fileValue) {
const classes = {}; // Map that points the old name to the new one.
const classRegex = /(\.([a-zA-Z_-]{1}[\w-_]+))/g; // Naive regex to match everything that start with dot.
const scopedFileValue = fileValue.replace(classRegex, (name) => scope(name, this.resourcePath, classes)); // Replace the old class with the new one and add it to the classes object
// Change the fileValue to scopedFileValue and export the classes.
return `
(${appendStyle.toString()})(${JSON.stringify(scopedFileValue)})
export default ${JSON.stringify(classes)}
` // Export allows the user to use it in his javascript code
}
```
In the `index.js`, we can now use it as an object:
```js
import React from 'react';
import { render } from "react-dom";
import classes from './index.css'; // Import the classes object.
render(
<div className={classes.app}>
Hello World
</div>,
document.getElementById('root')
)
```
Now it works with namespaced selector 🎉\
\
<mark>Some important points about the changes we implemented.</mark>
* When the loader is used by webpack, the context will be the loader context (`this`) from webpack. You can read more about it [here](https://webpack.js.org/api/loaders/). It provides the resolved file path, which makes the namespace unique to the file.
* The way we extract the classes selectors from the CSS file is a naive implementation that isn't taking into account other use cases. The ideal way is to use a CSS parser.
* `this.resourcePath` refers to the local path, which means that in other machines, the path may look different.
The loader is now implemented, and we've got scoped classes at this point. However, everything is loaded from JavaScript, and so it is not yet possible to cache the CSS.
To do this, we will need to compose all the CSS into one file, and to do that, we will need to create a webpack plugin.
## [Next chapter](./css-plugin.md)
### [Previous chapter](./setup-the-solution.md)
<file_sep># CSS Webpack Plugin - Style the modern web
_Styling a modern application is no simple task - traditionally it is done by serving HTML with CSS for styling, and sprinkling the web app with Javascript to get the job done.
The question is how to modernize the approach of setting up an app, and the answer is to use a bundler like Webpack and JavaScript framework / library like React. But how do we handle the **CSS**, and why is it not that simple as you would expect?_
## Agenda -
* [Part 1: Understanding the issue with native CSS.](./docs/native-css-issue.md)
* [Part 2: Setting up our webpack application without a CSS plugin.](./docs/setup-the-solution.md)
* [Part 3: Writing the Loader.](./docs/css-loader.md)
* [Part 4: Writing advanced Plugin.](./docs/css-plugin.md)
> If you are here just for implementation information, skip to part 3.
> Disclaimer - This is not a production-ready plugin. To see one that is, check out what my team and I are working on: [Stylable](https://stylable.io/)
## Development
This project is written as a Yarn workspace with TypeScript, and contains two main packages:
```
- css-loader
- css-plugin
```
For examples of different frameworks, see [`examples`](./examples).
### Bootstrap
```bash
yarn
yarn build
```
### Testing
The package `css-loader` has integration tests to ensure the correct output is received from the loader.
Inside the `css-plugin` package is an e2e test that creates a project, serves it with playwright, and makes sure that the UI works according to the output.
<file_sep>import { createApp } from 'vue';
import App from './app.vue';
const root = document.createElement('div')
root.id = 'root'
document.body.appendChild(root);
createApp(App).mount('#root')<file_sep>import { cssLoader } from './loader'
export * from './types';
export default cssLoader<file_sep>export const transformCSSClasses = {
css: `.root { color: red; } div .label { font-size: 1rem; }`,
expectedCSS: `.s7ad3f4__root { color: red; } div .s7ad3f4__label { font-size: 1rem; }`,
expected: `\n\n export default {"root":"s7ad3f4__root","label":"s7ad3f4__label"}`,
} as const
export const transformAndAddStyleScript = {
css: `.root { color: red; } div .label { font-size: 1rem; }`,
expected: `(function appendStyle(css) {\n const style = document.createElement('style');\n style.textContent = css;\n document.head.appendChild(style);\n})(".s7ad3f4__root { color: red; } div .s7ad3f4__label { font-size: 1rem; }")\n\n export default {"root":"s7ad3f4__root","label":"s7ad3f4__label"}`,
} as const
<file_sep>import { expect } from 'chai'
import { BASE_URL, getSimpleProject } from '../fixtures/simple-project'
describe('simple-project', () => {
const project = getSimpleProject().beforeAndAfter()
it('should serve simple project and find only css links tags', async () => {
await project.startProject({ waitMatcher: new RegExp(`Accepting connections at ${BASE_URL}`) })
const web = await project.initWebDriver()
await web.goto(BASE_URL)
expect(await web.styles()).to.deep.equal([])
expect(await web.links()).to.deep.equal([{ href: `${BASE_URL}/main.css`, rel: 'stylesheet' }])
})
})
<file_sep>export function appendStyle(css: string) {
const style = document.createElement('style')
style.textContent = css;
document.head.appendChild(style)
}<file_sep>import cssLoader from '@webpack-css/loader'
import { expect } from 'chai'
import { loaderContext, loaderContextWithoutOutputCSS } from './mocks'
import { transformCSSClasses, transformAndAddStyleScript } from './fixtures/css-loader'
describe('cssLoader', () => {
let ctx: any
beforeEach(() => {
ctx = loaderContext as any
})
it('should transform css classes', () => {
const { expected, css, expectedCSS } = transformCSSClasses;
expect(cssLoader.call(ctx, css)).to.equal(expected)
expect(loaderContext.setOutputCSS).to.have.been.calledWith(expectedCSS)
})
it('should transform and add style script', () => {
ctx = loaderContextWithoutOutputCSS
const { expected, css } = transformAndAddStyleScript;
expect(cssLoader.call(ctx, css)).to.equal(expected)
expect(loaderContext.setOutputCSS).to.not.have.been.called
})
})
<file_sep>{
"extends": "./tsconfig.base.json",
"files": [],
"compilerOptions": {
"types": [
"node",
"mocha",
"chai",
]
},
"references": [
{
"path": "./packages/css-loader/src"
},
{
"path": "./packages/css-loader/test"
},
{
"path": "./packages/css-plugin/src"
},
{
"path": "./packages/css-plugin/test"
}
]
}<file_sep># Example project Vue<file_sep>import type { WebpackPluginInstance, Compiler, Compilation, NormalModule } from 'webpack'
import type { ExtendedLoaderContext } from '@webpack-css/loader'
import type { ICSSPluginOptions } from './types'
import { injectLoader } from './inject-loader'
export class CSSPlugin implements WebpackPluginInstance {
private compiler!: Compiler
private cssMap = new Map<string, string>()
private options?: Required<ICSSPluginOptions>
constructor(
private readonly usersOptions: ICSSPluginOptions = {},
private readonly injectLoader = true
) { }
apply(compiler: Compiler) {
this.compiler = compiler;
if (this.injectLoader) {
injectLoader(this.compiler)
}
this.compiler.hooks.afterPlugins.tap(CSSPlugin.name, this.createOptions.bind(this, this.usersOptions))
this.compiler.hooks.thisCompilation.tap(CSSPlugin.name, this.handleCompilation.bind(this))
}
private handleCompilation(compilation: Compilation) {
this.compiler.webpack.NormalModule.getCompilationHooks(compilation).loader.tap(
CSSPlugin.name,
this.handleLoader.bind(this)
)
compilation.hooks.processAssets.tap(
{
name: CSSPlugin.name,
stage: this.compiler.webpack.Compilation.PROCESS_ASSETS_STAGE_DERIVED
},
this.handleProcessAssets.bind(this, compilation)
)
}
private handleLoader(webpackLoaderContext: object, module: NormalModule) {
const ctx = webpackLoaderContext as ExtendedLoaderContext<any>;
if (this.getOptions().inject === 'css') {
ctx.setOutputCSS = (css: string) => {
this.cssMap.set(module.resource, css)
}
}
}
private handleProcessAssets(compilation: Compilation) {
if (this.getOptions().inject !== 'css') return;
let assetName: string = this.getOptions().filename!
for (const [name, entry] of compilation.entrypoints) {
assetName ||= `${name}.css`
entry.getEntrypointChunk().files.add(assetName)
}
const asset = new this.compiler.webpack.sources.RawSource(this.getStaticCSS(), false)
compilation.emitAsset(assetName, asset)
}
private getStaticCSS() {
let raw = ''
for (const [path, css] of this.cssMap) {
raw += `/* ${path} */\n${css}\n`
}
return raw
}
private createOptions(defaults: ICSSPluginOptions) {
this.options = {
inject: defaults.inject ?? this.compiler.options.mode === 'production' ? 'css' : 'js',
filename: defaults.filename || ''
}
}
private getOptions() {
return this.options || this.usersOptions
}
}
<file_sep>export interface ICSSPluginOptions {
inject?: 'css' | 'js'
filename?: string
}<file_sep>import { mkdir, writeFile } from 'fs/promises'
import { resolve } from 'path'
export async function createFiles(rootDir: string, files: Dir) {
await mkdir(rootDir, { recursive: true })
for (const [fileName, fileValue] of Object.entries(files)) {
const currentPath = resolve(rootDir, fileName)
if (typeof fileValue === 'string') {
await writeFile(currentPath, fileValue)
} else if (Boolean(fileValue) && typeof fileValue === 'object') {
await createFiles(currentPath, fileValue)
}
}
}
export type Dir = {
[name: string]: string | Dir
}<file_sep>export * from './create-files'
export * from './exec'
export * from './get-package'
export * from './setup-test'<file_sep>export const getPackageJSON = (defaults: object) => JSON.stringify(({
...defaults,
"name": "example" + Math.random().toString(36).slice(2),
"version": "1.0.0",
"main": "index.js",
"private": true,
"license": "MIT",
"scripts": {
"clean": "rm -rf dist",
"start": "webpack serve --open",
"build": "webpack --mode=production --node-env=production",
"build:dev": "webpack --mode=development",
"build:prod": "webpack --mode=production --node-env=production",
"watch": "webpack --watch",
"serve": "serve ./dist -l 5000"
},
"dependencies": {
"react": "^17.0.2",
"react-dom": "^17.0.2"
}
}))<file_sep>import { Dir, getPackageJSON, setup } from '../utils';
export const BASE_URL = 'http://localhost:5000';
export const getSimpleProject = () => {
const name = 'simple-project';
const files: Dir = {
'package.json': getPackageJSON({ name }),
src: {
'index.css': `.root { background: red; }`,
'index.tsx': `
import classes from './index.css';
import ReactDOM from 'react-dom';
ReactDOM.render(
<div className={classes.root} >Hello</div>,
document.body.appendChild(document.createElement('main'))
)
`
},
'webpack.config.js': `
const HtmlWebpackPlugin = require('html-webpack-plugin');
const { CSSPlugin } = require('@webpack-css/plugin')
module.exports = {
mode: 'development',
devtool: 'source-map',
entry: './src/index.tsx',
module: {
rules: [
{
test: /.tsx?$/,
loader: 'ts-loader',
options: {
transpileOnly: true
}
},
{
test: /.(png|jpg|jpeg|gif|svg)$/,
type: 'asset',
},
],
},
resolve: {
extensions: ['ts', 'tsx', '.js', '.json'],
},
plugins: [new CSSPlugin(), new HtmlWebpackPlugin()],
};
`
}
return setup({ files })
}<file_sep>
# Understanding the issue with native CSS.
## Our options
Native CSS is implemented in different ways:
* The first and the most simple way to include CSS is using inline styling, which means that you explicitly include a style in an HTML tag. `<span style="color:red;">...</span>`
* A different solution is to use an HTML tag called `<style>...</style>`, where its text content is the style itself, and it is used to select the different HTML elements.
* Another option is to load a CSS file via a link tag, and select the different HTML elements inside the file.
## The problems
Each one of the solutions above has its benefits and tradeoffs. It is very important to understand them to avoid unexpected behavior in your styling. You'll find that none of those solutions, however, solve one of the most problematic issues - that **CSS is global**.
The global issue is a pretty difficult one to overcome. Let's say you have a button with a class called `btn` and you style it. One day your co-worker works on a different page that has a button too, and he also decided to call it `btn`. The problem should be apparent - the styles would clash.
Another significant issue is **specificity**, where the specificity is equal between selectors, and the last declaration found in the CSS is applied to the element. To put it simply - your order matters.
## [Next chapter](./setup-the-solution.md)<file_sep>declare module '*.css' {
const defaultExport: Record<string, string>;
export default defaultExport;
}<file_sep>import { createHash } from 'crypto'
export function resolveNamespace(filepath: string) {
const h = createHash('sha1')
h.write(filepath)
return 's' + h.digest('hex').slice(0, 6)
}<file_sep>module.exports = {
'enable-source-maps': true,
file: ['./mocha.setup.ts'],
timeout: 20000
};<file_sep>import { transform } from './transform';
import { appendStyle } from "./append-style";
import type { ExtendedLoaderContext } from './types';
export function cssLoader(this: ExtendedLoaderContext<any>, content: string): string {
let injectCssInJs = true
const { classesMap, css } = transform(this.resourcePath, content)
if (this.setOutputCSS) {
injectCssInJs = false
this.setOutputCSS(css)
}
return `${injectCssInJs ? `(${appendStyle})(${JSON.stringify(css)})` : ''}
export default ${JSON.stringify(Object.fromEntries(classesMap.entries()))}`
}<file_sep>import postcss from 'postcss'
import selectorParser from 'postcss-selector-parser'
import { resolveNamespace } from './resolve-namespace'
export function transform(path: string, content: string) {
const process = postcss.parse(content, { from: path })
const classesMap = new Map()
process.walkRules(node => {
const ruleAst = selectorParser().astSync(node);
ruleAst.walkClasses(selectorAst => {
if (!classesMap.has(selectorAst.value)) {
classesMap.set(selectorAst.value, `${resolveNamespace(path)}__${selectorAst.value}`)
}
selectorAst.value = classesMap.get(selectorAst.value)
})
node.selector = ruleAst.toString()
})
const css = process.toString()
return {
css,
classesMap
}
}<file_sep>import type { Browser, Page, BrowserContext } from 'playwright';
export class Driver {
private page!: Page
private context!: BrowserContext
currentUrl: string | undefined
private constructor(
private browser: Browser
) { }
static create(browser: Browser) {
return new this(browser).init()
}
async init() {
this.context = await this.browser.newContext()
this.page = await this.context.newPage()
return this
}
async goto(url: string) {
this.currentUrl = url;
return this.page.goto(url)
}
async links() {
return await this.page.$$eval('link', links => links.map(link => ({
href: link.href,
rel: link.rel
})))
}
async styles() {
return await this.page.$$eval('style', styles => styles.map(s => s.textContent))
}
}<file_sep># Setting up our webpack application without a CSS plugin.
## The solutions
Currently, there are many different solutions to these problems, from utility frameworks, CSS preprocessors, and others that all try to help with the issues that native CSS has.
In this article, I would like to solve some of those problems from scratch with you.
Let's set up our environment real quick. To do this, run these commands:
```bash
mkdir example-css-plugin
cd example-css-plugin
npm init -y
npm i -D webpack webpack-cli @webpack-cli/generators @babel/preset-react
npm i react react-dom
```
When the development dependencies have finished installing, run the webpack init command:
```bash
npx webpack init
```
For our setup, your answers should look like this:
```
? Which of the following JS solutions do you want to use? ES6
? Do you want to use webpack-dev-server? Yes
? Do you want to simplify the creation of HTML files for your bundle? Yes
? Do you want to add PWA support? No
? Which of the following CSS solutions do you want to use? none
? Do you like to install prettier to format generated configuration? No
```
### **Make sure that this question is answered as such: "Which of the following CSS solutions do you want to use" - none.**
## Configure React
Go to `.babelrc` and make sure that the presets array includes "@babel/preset-react".
```json
{
"plugins": ["@babel/syntax-dynamic-import"],
"presets": [
[
"@babel/preset-env",
{
"modules": false
}
],
"@babel/preset-react"
]
}
```
Now we need to go to `index.html` and make sure it has div with root id.
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title>CSS Webpack Plugin example</title>
</head>
<body>
<div id="root"></div>
</body>
</html>
```
After all of that, we are ready to write our app inside `src/index.js`:
```js
import React from 'react';
import { render } from "react-dom";
render(
<div>
Hello World
</div>,
document.getElementById('root')
)
```
## [Next chapter](./css-loader.md)
### [Previous chapter](./native-css-issue.md)<file_sep>
# Writing an advanced Plugin
As said, we implemented a loader that can inject CSS into our page. What if we want to do it with a signal file and not an injection, however?
Loading CSS as a file comes with many benefits, and the best of them is caching. The browser can cache the file and won't need to redownload it every time it is needed.
This operation is more complicated than the loader case since we will have more context about the webpack bundling process.
### What is a plugin?
*A webpack plugin is a JavaScript object that has an [apply](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Function/apply) method. This apply method is called by the webpack compiler, giving access to the entire compilation lifecycle.*
## Creating the Plugin
Let's create a file called `plugin.js`, and create the plugin skeleton:
```js
class CSSPlugin {
cssMap = new Map() // We will save here the CSS content
/**
* Hook into the compiler
* @param {import('webpack').Compiler} compiler
*/
apply(compiler) { }
}
module.exports = {
CSSPlugin
}
```
Now let's implement the apply method:
```js
class CSSPlugin {
cssMap = new Map() // We will save here the CSS content
/**
* Hook into the compiler
* @param {import('webpack').Compiler} compiler
*/
apply(compiler) {
// Hook into the global compilation.
compiler.hooks.thisCompilation.tap('CSSPlugin', (compilation) => {
// Hook into the loader to save the CSS content.
compiler.webpack.NormalModule.getCompilationHooks(compilation).loader.tap(
'CSSPlugin',
(context, module) => {
// Setting up a method on the loader context that we will use inside the loader.
context.setOutputCSS = (css) => {
// the key is the resource path, and the CSS is the actual content.
this.cssMap.set(module.resource, css)
}
}
)
})
}
}
```
We hooked into the global compilation and then hooked into the loader (which was implemented in the previous chapter).
When the loader content is reachable, we add the `setOutputCSS` method to call it from the loader.
Here's how to call this method in `loader.js`:
```js
function loader(fileValue) {
const classes = {}; // Map that points the old name to the new one.
const classRegex = /(\.([a-zA-Z_-]{1}[\w-_]+))/g; // Naive regex to match everything that start with dot.
const scopedFileValue = fileValue.replace(classRegex, (name) => scope(name, this.resourcePath, classes)); // Replace the old class with the new one and add it to the classes object
this.setOutputCSS(scopedFileValue) // Pass the scoped CSS output
// Export the classes.
return `export default ${JSON.stringify(classes)}`
}
```
As you can see, we are not appending the style in the JavaScript. We use the method we added to the context.
After collecting all the scoped CSS content, we now need to hook into the asset process hook to let the compiler know that we have a new asset that it should handle.
Let's add it to the `apply` method:
```js
class CSSPlugin {
// ...
apply(compiler) {
compiler.hooks.thisCompilation.tap(
'CSSPlugin',
(compilation) => {
// ...
// Hook into the process assets hook
compilation.hooks.processAssets.tap(
{
name: 'CSSPlugin',
stage: compiler.webpack.Compilation.PROCESS_ASSETS_STAGE_DERIVED
},
() => {
// Loop over the CSS content and add it to the content variable
let content = '';
for (const [path, css] of this.cssMap) {
content += `/* ${path} */\n${css}\n`;
}
// Append the asset to the entries.
for (const [name, entry] of compilation.entrypoints) {
assetName = `${name}.css`;
entry.getEntrypointChunk().files.add(assetName);
}
// Create the source instance with the content.
const asset = new compiler.webpack.sources.RawSource(content, false);
// Add it to the compilation
compilation.emitAsset(assetName, asset);
}
)
}
}
```
Now we'll run the build command:
```bash
npm run build
```
We should see `main.css` in the output folder, and also injected into the HTML:\
Output:\
\
`index.html`:\
\
And that's it!\
We finished the Plugin and have one CSS file for all of the CSS.
Note that we skipped dependencies, graph ordering, and filtering unused CSS for demonstration purposes.
You can see my full implementation with typescript and tests, [here](../README.md) in this repo.
If you have any question you can reach me via [linkedin](https://www.linkedin.com/in/tzach-bonfil-21b822187/). I hope I managed to help you. Have a good one!
### [Previous chapter](./css-loader.md)
<file_sep>import { spawn, SpawnOptions } from 'child_process';
interface ExecOptions extends SpawnOptions {
processKiller?: Set<Function>
}
export function exec(command: string, options?: ExecOptions) {
let totalData = ''
let resolve: Function
const emit = () => new Promise((res, _rej) => {
options?.processKiller?.add(kill)
resolve = () => {
res(undefined)
process.kill()
};
const process = spawn(
command.split(' ').shift()!,
command.split(' ').slice(1),
options || {}
)
process.stderr!.on('data', (err: Buffer) => _rej(new Error(err.toString())))
process.stdout!.on('close', () => {
res(totalData)
options?.processKiller?.delete(kill)
})
process.stdout!.on('data', (data: Buffer) => {
totalData += data.toString()
})
})
const waitForOutput = (stringOrRegex: string | RegExp) => new Promise((res, rej) => {
const timeout = setTimeout(() => {
rej(new Error(`waitForOutput: Timeout waiting for ${stringOrRegex}`))
clearInterval(interval)
clearTimeout(timeout)
}, 10000);
const interval = setInterval(() => {
if (typeof stringOrRegex === 'string' ? totalData.includes(stringOrRegex) : stringOrRegex.test(totalData)) {
res(undefined)
clearInterval(interval)
clearTimeout(timeout)
}
}, 50)
})
const kill = () => {
resolve()
}
return {
emit,
kill,
waitForOutput
}
}<file_sep>import { dirname, resolve } from 'path'
import type { Browser } from 'playwright'
import rimraf from 'rimraf'
import { promisify } from 'util'
import { createFiles, Dir } from './create-files'
import { exec } from './exec'
import { Driver } from './page-driver'
interface SetupOptions {
files: Dir
}
export function setup(
{
files
}: SetupOptions
) {
let _driver: Driver | undefined;
const processKiller = new Set<Function>()
const rootDir = resolve(
dirname(dirname(dirname(require.resolve('@webpack-css/plugin')))),
`temp-${Math.random().toString(36).slice(2)}`
)
const beforeAndAfter = () => {
beforeEach(async () => {
await createFiles(rootDir, files)
})
afterEach(async () => {
await promisify(rimraf)(rootDir)
for (const kill of processKiller) {
kill()
}
})
return payload
}
const run = (command: 'build' | 'start' | 'test' | 'install' | 'serve') => exec(
`yarn ${command}`,
{ cwd: rootDir, processKiller }
)
const browser = () => (global as any)._browser as Browser
const startProject = async (
{
waitMatcher
}: {
waitMatcher?: string | RegExp
} = {}
) => {
await run('install').emit()
await run('build').emit();
const serveProcess = run('serve');
serveProcess.emit()
if (waitMatcher) {
await serveProcess.waitForOutput(waitMatcher)
}
}
const webDriver = () => {
return _driver
}
const initWebDriver = async () => {
_driver = await Driver.create(payload.browser())
return payload.webDriver()!
}
const payload = {
files,
rootDir,
beforeAndAfter,
run,
browser,
startProject,
processKiller,
webDriver,
initWebDriver
}
return payload
}
<file_sep>import type { Compiler } from 'webpack';
export function injectLoader(compiler: Compiler) {
if (!compiler.options.module.rules) {
compiler.options.module.rules = [];
}
compiler.options.module.rules.unshift({
test: /\.css$/,
loader: require.resolve('@webpack-css/loader'),
sideEffects: true,
});
}
<file_sep>const { CSSPlugin } = require('@webpack-css/plugin')
const { VueLoaderPlugin } = require("vue-loader");
const HtmlWebpackPlugin = require("html-webpack-plugin");
/** @type {import('webpack').Configuration} */
module.exports = {
module: {
rules: [
{
test: /\.vue$/,
loader: "vue-loader",
},
{
test: /\.svg$/,
type: 'asset'
},
],
},
plugins: [
new CSSPlugin(),
new VueLoaderPlugin(),
new HtmlWebpackPlugin(),
],
}; | 1b64782b646a25ce83f94123889fd268d6f16530 | [
"Markdown",
"TypeScript",
"JavaScript",
"JSON with Comments"
] | 33 | TypeScript | tzachbon/css-webpack-plugin-example | 34d7b496e58ed524d1940fd1e350e6ef24e21af7 | 188e8903631e0f1b2a6e5e1efe549b2297aaa21f | |
refs/heads/master | <file_sep># ase-assignment2
To run all tests, use `mvn verify` in the `endpoint` folder. To run the application, use `mvn thorntail:run`.
To regenerate pact file, use `mvn verify` in the `endpoint-client` folder.
To package as uber jar, use `mvn clean install package` in `endpoint` folder (+ `verify` to run pact tests while packaging), then `java -jar target/endpoint-thorntail.jar`).
<file_sep>package edu.baylor.ecs.endpoint.model;
import java.time.LocalDateTime;
public class TestPersonObject extends Person {
public TestPersonObject(Integer id) {
this.id = id;
}
TestPersonObject(Integer id,
String name,
Integer age,
String email,
Integer version) {
this.id = id;
this.name = name;
this.age = age;
this.email = email;
this.version = version;
}
}
<file_sep>-- id, age, email, name, version
INSERT INTO person VALUES(1, 30, '<EMAIL>', '<NAME>', 1);
INSERT INTO person VALUES(2, 24, '<EMAIL>', '<NAME>', 1);
INSERT INTO person VALUES(3, 56, '<EMAIL>', '<NAME>', 1);
INSERT INTO person VALUES(4, 19, '<EMAIL>', '<NAME>', 1);
INSERT INTO person VALUES(5, 41, '<EMAIL>', '<NAME>', 1);
-- id, brand, license plate, owner, type, version
INSERT INTO car VALUES(1, 'Chevrolet', 'ABC 123', 'Silverado', 1, 1);
INSERT INTO car VALUES(2, 'Chevrolet', 'PRD 845', 'Suburban', 1, 1);
INSERT INTO car VALUES(3, 'Nissan', 'HKJ3456', 'Ultima', 1, 2);
INSERT INTO car VALUES(4, 'Toyota', 'BTN4377', 'Highlander', 1, 3);
INSERT INTO car VALUES(5, 'Ford', 'FGR5591', 'Focus', 1, 3);
INSERT INTO car VALUES(6, 'Ford', 'OOP9191', 'F-150', 1, 3);
INSERT INTO car VALUES(7, 'Lamborghini', 'LKL0987', 'Murcielago', 1, 4);
INSERT INTO car VALUES(8, 'Ford', 'MBT2048', 'Taurus', 1, 4);
INSERT INTO car VALUES(9, 'Hyundai', 'BVB6655', 'Sonata', 1, 5);
INSERT INTO car VALUES(10, 'Chevrolet', 'OMG4242', 'Blazer', 1, 5);
INSERT INTO car VALUES(11, 'Chevrolet', 'LNB1234', 'Traverse', 1, 5);
<file_sep>package edu.baylor.ecs.endpoint;
import edu.baylor.ecs.endpoint.model.Car;
import edu.baylor.ecs.endpoint.model.Person;
import javax.enterprise.context.ApplicationScoped;
import javax.persistence.EntityManager;
import javax.persistence.PersistenceContext;
import javax.transaction.Transactional;
import javax.validation.ConstraintViolationException;
import javax.ws.rs.*;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.Response;
import java.net.URI;
import java.util.Collection;
import java.util.List;
@Path("/person")
@ApplicationScoped
public class PersonResource {
@PersistenceContext(unitName = "EndpointPU")
private EntityManager em;
@GET
@Path("/")
@Produces(MediaType.APPLICATION_JSON)
public Collection<Person> findAll() throws Exception {
return em.createNamedQuery("Person.findAll", Person.class)
.getResultList();
}
@GET
@Produces(MediaType.APPLICATION_JSON)
@Path("/{personId}")
public Response get(@PathParam("personId") Integer personId) {
Person person = em.find(Person.class, personId);
if (person == null) {
return Response
.status(Response.Status.NOT_FOUND)
.entity(null)
.build();
}
return Response
.status(Response.Status.OK)
.entity(person)
.build();
}
@POST
@Path("/")
@Consumes(MediaType.APPLICATION_JSON)
@Produces(MediaType.APPLICATION_JSON)
@Transactional
public Response create(Person person) throws Exception {
if (person.getId() != null) {
return Response
.status(Response.Status.CONFLICT)
.entity("Unable to create Person, id was already set.")
.build();
}
try {
em.persist(person);
em.flush();
} catch (ConstraintViolationException e) {
return Response
.status(Response.Status.BAD_REQUEST)
.entity("Bad entity!")
.build();
} catch (Exception e) {
return Response
.serverError()
.entity(e.getMessage())
.build();
}
return Response
.created(new URI("person/" + person.getId().toString()))
.build();
}
@DELETE
@Produces(MediaType.APPLICATION_JSON)
@Path("/{personId}")
@Transactional
public Response remove(@PathParam("personId") Integer personId) throws Exception {
try {
Person person = em.find(Person.class, personId);
List<Car> cars = em.createNamedQuery("Car.findAllByPerson")
.setParameter("owner", person)
.getResultList();
// get rid of ownership for this person, but don't delete the cars
for (Car car : cars) {
car.setOwner(null);
em.merge(car);
}
em.remove(person);
} catch (Exception e) {
return Response
.serverError()
.entity(e.getMessage())
.build();
}
return Response
.noContent()
.build();
}
@PUT
@Consumes(MediaType.APPLICATION_JSON)
@Produces(MediaType.APPLICATION_JSON)
@Path("/{personId}")
@Transactional
public Response update(@PathParam("personId") Integer personId, Person person) throws Exception {
try {
Person entity = em.find(Person.class, personId);
if (null == entity) {
return Response
.status(Response.Status.NOT_FOUND)
.entity("Person with id of " + personId + " does not exist.")
.build();
}
if (person.getId() == null || !person.getId().equals(personId)) {
return Response
.status(Response.Status.BAD_REQUEST)
.entity("Person id expected to be " + personId + ", but was " + person.getId() + ".")
.build();
}
// all this setter logic would go in a service method, if there was a service layer
entity.setName(person.getName());
entity.setEmail(person.getEmail());
entity.setAge(person.getAge());
try {
Person merged = em.merge(entity);
em.flush();
return Response
.ok(merged)
.build();
} catch (ConstraintViolationException e) {
return Response.status(Response.Status.BAD_REQUEST)
.entity("Bad entity!")
.build();
}
} catch (Exception e) {
return Response
.serverError()
.entity(e.getMessage())
.build();
}
}
@GET
@Produces(MediaType.APPLICATION_JSON)
@Path("/{personId}/cars")
public Response getCars(@PathParam("personId") Integer personId) {
Person person = em.find(Person.class, personId);
if (person == null) {
return Response
.status(Response.Status.NOT_FOUND)
.entity("Person with id of " + personId + " does not exist.")
.build();
}
List<Car> cars = em.createNamedQuery("Car.findAllByPerson")
.setParameter("owner", person)
.getResultList();
return Response
.status(Response.Status.OK)
.entity(cars)
.build();
}
}
<file_sep>package edu.baylor.ecs.endpoint;
import java.util.List;
import java.util.Map;
import edu.baylor.ecs.endpoint.model.Person;
import edu.baylor.ecs.endpoint.model.TestPersonObject;
import io.restassured.RestAssured;
import io.restassured.http.ContentType;
import io.restassured.path.json.JsonPath;
import io.restassured.response.Response;
import org.jboss.arquillian.container.test.api.RunAsClient;
import org.jboss.arquillian.junit.Arquillian;
import org.junit.BeforeClass;
import org.junit.FixMethodOrder;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.junit.runners.MethodSorters;
import org.wildfly.swarm.arquillian.DefaultDeployment;
import static io.restassured.RestAssured.given;
import static io.restassured.RestAssured.when;
import static org.fest.assertions.Assertions.assertThat;
@RunWith(Arquillian.class)
@DefaultDeployment
@RunAsClient
@FixMethodOrder(MethodSorters.NAME_ASCENDING)
public class PersonResourceTest {
@BeforeClass
public static void setup() throws Exception {
RestAssured.baseURI = "http://localhost:8080";
}
@Test
public void testGetAllPersons() throws Exception {
Response response =
when()
.get("/person")
.then()
.extract().response();
String jsonAsString = response.asString();
List<Map<String, ?>> jsonAsList = JsonPath.from(jsonAsString).getList("");
// size of list
assertThat(jsonAsList.size()).isEqualTo(5);
Map<String, ?> record1 = jsonAsList.get(0);
// spot checking values
assertThat(record1.get("id")).isEqualTo(1);
assertThat(record1.get("name")).isEqualTo("<NAME>");
assertThat(record1.get("age")).isEqualTo(30);
Map<String, ?> record2 = jsonAsList.get(2);
assertThat(record2.get("id")).isEqualTo(3);
assertThat(record2.get("name")).isEqualTo("<NAME>");
assertThat(record2.get("age")).isEqualTo(56);
}
@Test
public void testGetPerson() throws Exception {
Response response =
given()
.pathParam("personId", 2)
.when()
.get("/person/{personId}")
.then()
.extract().response();
String jsonAsString = response.asString();
Person person = JsonPath.from(jsonAsString).getObject("", Person.class);
assertThat(person.getId()).isEqualTo(2);
assertThat(person.getName()).isEqualTo("<NAME>");
assertThat(person.getAge()).isEqualTo(24);
}
@Test
public void testCreatePerson() throws Exception {
Person newPerson = new Person();
newPerson.setName("<NAME>");
newPerson.setAge(92);
newPerson.setEmail("<EMAIL>");
Response response =
given()
.contentType(ContentType.JSON)
.body(newPerson)
.when()
.post("/person");
assertThat(response).isNotNull();
assertThat(response.getStatusCode()).isEqualTo(201);
String locationUrl = response.getHeader("Location");
Integer personId = Integer.valueOf(locationUrl.substring(locationUrl.lastIndexOf('/') + 1));
response =
when()
.get("/person")
.then()
.extract().response();
String jsonAsString = response.asString();
List<Map<String, ?>> jsonAsList = JsonPath.from(jsonAsString).getList("");
assertThat(jsonAsList.size()).isEqualTo(6);
response =
given()
.pathParam("personId", personId)
.when()
.get("/person/{personId}")
.then()
.extract().response();
jsonAsString = response.asString();
Person person = JsonPath.from(jsonAsString).getObject("", Person.class);
assertThat(person.getId()).isEqualTo(personId);
assertThat(person.getName()).isEqualTo("<NAME>");
assertThat(person.getAge()).isEqualTo(92);
assertThat(person.getEmail()).isEqualTo("<EMAIL>");
}
@Test
public void testCreatePersonNullField() throws Exception {
Person sirNoName = new Person();
sirNoName.setEmail("<EMAIL>");
sirNoName.setAge(49);
Response response =
given()
.contentType(ContentType.JSON)
.body(sirNoName)
.when()
.post("/person");
assertThat(response).isNotNull();
assertThat(response.getStatusCode()).isEqualTo(400);
}
@Test
public void testUpdatePerson() {
Person rob = new TestPersonObject(4);
rob.setName("<NAME>");
rob.setAge(19);
rob.setEmail("<EMAIL>"); // he ditched yahoo
Response response =
given()
.contentType(ContentType.JSON)
.pathParam("personId", 4)
.body(rob)
.when()
.put("/person/{personId}");
assertThat(response).isNotNull();
assertThat(response.getStatusCode()).isEqualTo(200);
response =
given()
.pathParam("personId", 4)
.when()
.get("/person/{personId}")
.then()
.extract().response();
String jsonAsString = response.asString();
Person person = JsonPath.from(jsonAsString).getObject("", Person.class);
assertThat(person.getId()).isEqualTo(4);
assertThat(person.getEmail()).isEqualTo("<EMAIL>");
}
@Test
public void testUpdatePersonNullId() {
Person rob = new Person(); // no ID
rob.setName("<NAME>");
rob.setAge(19);
rob.setEmail("<EMAIL>");
Response response =
given()
.contentType(ContentType.JSON)
.pathParam("personId", 4)
.body(rob)
.when()
.put("/person/{personId}");
assertThat(response).isNotNull();
assertThat(response.getStatusCode()).isEqualTo(400);
response =
given()
.pathParam("personId", 4)
.when()
.get("/person/{personId}")
.then()
.extract().response();
String jsonAsString = response.asString();
Person person = JsonPath.from(jsonAsString).getObject("", Person.class);
assertThat(person.getId()).isEqualTo(4);
// assert email didn't change
assertThat(person.getName()).isEqualTo("<NAME>");
}
@Test
public void testDeletePerson() {
// get list as is
Response response =
when()
.get("/person")
.then()
.extract().response();
String oldJsonString = response.asString();
List<Map<String, ?>> oldList = JsonPath.from(oldJsonString).getList("");
response =
given()
.pathParam("personId", 5)
.when()
.delete("/person/{personId}")
.then()
.extract().response();
assertThat(response.getStatusCode()).isEqualTo(204);
// get the new list
response =
when()
.get("/person")
.then()
.extract().response();
String newJsonString = response.asString();
List<Map<String, ?>> newList = JsonPath.from(newJsonString).getList("");
assertThat(newList.size()).isEqualTo(oldList.size() - 1);
// try to get the deleted person
response =
given()
.pathParam("personId", 5)
.when()
.get("/person/{personId}")
.then()
.extract().response();
assertThat(response.getStatusCode()).isEqualTo(404);
}
}
<file_sep>package edu.baylor.ecs.endpoint.model;
import com.fasterxml.jackson.annotation.JsonIdentityInfo;
import com.fasterxml.jackson.annotation.ObjectIdGenerators;
import javax.persistence.*;
import javax.validation.constraints.NotNull;
import javax.validation.constraints.Pattern;
import javax.validation.constraints.Size;
import java.time.LocalDateTime;
import java.util.Objects;
@Entity
@Table(name = "person")
@NamedQueries({
@NamedQuery(name = "Person.findAll", query = "select p from Person p")
})
@JsonIdentityInfo(generator = ObjectIdGenerators.PropertyGenerator.class, property = "id", scope = Person.class)
public class Person {
@Id
@SequenceGenerator(
name = "person_sequence",
allocationSize = 1,
initialValue = 6
)
@GeneratedValue(strategy = GenerationType.SEQUENCE, generator = "person_sequence")
@Column(name = "id", updatable = false, nullable = false)
protected Integer id;
@NotNull
@Size(min = 3, max = 50)
@Column(nullable = false)
protected String name;
@NotNull
@Column(nullable = false)
@Pattern(regexp = "^[A-Z0-9._%+-]+@[A-Z0-9.-]+\\.[A-Z]{2,}$", flags = Pattern.Flag.CASE_INSENSITIVE)
protected String email;
@Column(nullable = false)
protected int age;
@Version
protected Integer version = 0;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public Integer getVersion() {
return version;
}
public void setVersion(Integer version) {
this.version = version;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Person other = (Person) o;
// person ID not included in equality check
return Objects.equals(name, other.name) &&
Objects.equals(email, other.email) &&
Objects.equals(age, other.age) &&
Objects.equals(version, other.version);
}
@Override
public int hashCode() {
// person ID not included in hash code
return Objects.hash(age, email, name, version);
}
}
| 1e4f5d288a73147042e4820bada5806fb543374d | [
"Markdown",
"Java",
"SQL"
] | 6 | Markdown | vinbush/ase-assignment2 | a4c3686860600321d95abd8ea128d9cb5022a114 | 8869186597b6a84075354201e207addc07b03427 | |
refs/heads/main | <file_sep>/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package modeli;
import domen.Plesac;
import java.util.List;
import javax.swing.table.AbstractTableModel;
/**
*
* @author Nada
*/
public class ModelTabelePlesac extends AbstractTableModel{
private List<Plesac> lista;
private final String[] kolone;
public ModelTabelePlesac(List<Plesac> lista) {
this.lista = lista;
this.kolone = new String[] {"id", "ime", "prezime", "broj godina", "pozicija"};
}
@Override
public int getRowCount() {
return lista.size();
}
@Override
public int getColumnCount() {
return kolone.length;
}
@Override
public Object getValueAt(int rowIndex, int columnIndex) {
Plesac pl = lista.get(rowIndex);
switch(columnIndex){
case 0: return pl.getPlesacID()+"";
case 1: return pl.getIme();
case 2: return pl.getPrezime();
case 3: return pl.getBrojGodina()+"";
case 4: return pl.getPozicija();
default: return "N/A";
}
}
@Override
public String getColumnName(int column) {
return kolone[column];
}
public Plesac getRed(int i) {
return lista.get(i);
}
public void setLista(List<Plesac> lista) {
this.lista = lista;
fireTableDataChanged();
}
}
<file_sep>/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package db;
import domen.IOpstiDomenskiObjekat;
import helpers.PropertyReader;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.List;
import konstante.Konstante;
/**
*
* @author Nada
*/
public class DBBroker {
Connection konekcija;
PropertyReader pr;
private static DBBroker instance;
private DBBroker() {
pr = new PropertyReader();
}
public static DBBroker getInstance() {
if(instance == null)
instance = new DBBroker();
return instance;
}
public void ucitajDrajver() throws ClassNotFoundException{
Class.forName(pr.readForKey(Konstante.DRIVER));
}
public void otvoriKonekciju() throws SQLException{
konekcija = DriverManager.getConnection(pr.readForKey(Konstante.URL), pr.readForKey(Konstante.USER), pr.readForKey(Konstante.PASSWORD));
konekcija.setAutoCommit(false);
}
public void zatvoriKonekciju() throws SQLException{
konekcija.close();
}
public void commit() throws SQLException{
konekcija.commit();
}
public void rollback() throws SQLException{
konekcija.rollback();
}
public List<IOpstiDomenskiObjekat> select(IOpstiDomenskiObjekat odo) throws SQLException{
String upit = "SELECT * FROM "+odo.nazivTabele()+" "+odo.alijas()+" "+odo.join()+" "+odo.selectWhere();
System.out.println(upit);
Statement s = konekcija.createStatement();
ResultSet rs = s.executeQuery(upit);
return odo.ucitajListu(rs);
}
public void insert(IOpstiDomenskiObjekat odo) throws SQLException{
String upit = "INSERT INTO "+odo.nazivTabele()+" "+odo.koloneInsert()+" VALUES("+odo.vrednostiInsert()+")";
System.out.println(upit);
Statement s = konekcija.createStatement();
s.executeUpdate(upit);
}
public void update(IOpstiDomenskiObjekat odo) throws SQLException{
String upit = "UPDATE "+odo.nazivTabele()+" SET "+odo.vrednostiUpdate()+" WHERE "+odo.vrednostPrimarniKljuc();
System.out.println(upit);
Statement s = konekcija.createStatement();
s.executeUpdate(upit);
}
public void delete(IOpstiDomenskiObjekat odo) throws SQLException{
String upit = "DELETE FROM "+odo.nazivTabele()+" WHERE "+odo.vrednostPrimarniKljuc();
System.out.println(upit);
Statement s = konekcija.createStatement();
s.executeUpdate(upit);
}
public long max(IOpstiDomenskiObjekat odo) throws SQLException{
String upit ="SELECT max("+odo.vratiMax()+") as max from "+odo.nazivTabele();
System.out.println(upit);
Statement s = konekcija.createStatement();
ResultSet rs = s.executeQuery(upit);
long max = 0l;
while (rs.next()) {
max = rs.getLong("max");
}
return max;
}
}
<file_sep>/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package niti;
import domen.IOpstiDomenskiObjekat;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.net.Socket;
import java.util.logging.Level;
import java.util.logging.Logger;
import konstante.Operacije;
import kontroler.Kontroler;
import transfer.KlijentskiZahtev;
import transfer.ServerskiOdgovor;
/**
*
* @author Nada
*/
public class ObradaZahtevaNit extends Thread {
Socket s;
boolean kraj = false;
public ObradaZahtevaNit(Socket s) {
this.s = s;
}
@Override
public void run() {
while (!kraj) {
KlijentskiZahtev kz = primiZahtev();
ServerskiOdgovor so = new ServerskiOdgovor();
System.out.println("Operacija: " + kz.getOperacija());
switch (kz.getOperacija()) {
case Operacije.PRIJAVA:
so = Kontroler.getInstance().prijava((IOpstiDomenskiObjekat)kz.getParametar());
break;
case Operacije.NOVI_PLESAC:
so = Kontroler.getInstance().noviPlesac((IOpstiDomenskiObjekat)kz.getParametar());
break;
case Operacije.IZMENA_PLESACA:
so = Kontroler.getInstance().izmenaPlesaca((IOpstiDomenskiObjekat)kz.getParametar());
break;
case Operacije.BRISANJE_PLESACA:
so = Kontroler.getInstance().brisanjePlesaca((IOpstiDomenskiObjekat)kz.getParametar());
break;
case Operacije.PRETRAGA_PLESACA:
so = Kontroler.getInstance().pretragaPlesaca((IOpstiDomenskiObjekat)kz.getParametar());
break;
case Operacije.UCITAVANJE_PLESACA:
so = Kontroler.getInstance().ucitavanjePlesaca((IOpstiDomenskiObjekat)kz.getParametar());
break;
case Operacije.NOVO_TAKMICENJE:
so = Kontroler.getInstance().novoTakmicenje((IOpstiDomenskiObjekat)kz.getParametar());
break;
case Operacije.IZMENA_TAKMICENJA:
so = Kontroler.getInstance().izmenaTakmicenja((IOpstiDomenskiObjekat)kz.getParametar());
break;
case Operacije.BRISANJE_TAKMICENJA:
so = Kontroler.getInstance().brisanjeTakmicenja((IOpstiDomenskiObjekat)kz.getParametar());
break;
case Operacije.PRETRAGA_TAKMICENJA:
so = Kontroler.getInstance().pretragaTakmicenja((IOpstiDomenskiObjekat)kz.getParametar());
break;
case Operacije.UCITAVANJE_TAKMICENJA:
so = Kontroler.getInstance().ucitavanjeTakmicenja((IOpstiDomenskiObjekat)kz.getParametar());
break;
case Operacije.UCITAVANJE_POZICIJA:
so = Kontroler.getInstance().ucitavanjePozicija();
}
posaljiOdgovor(so);
}
}
private KlijentskiZahtev primiZahtev() {
KlijentskiZahtev kz = new KlijentskiZahtev();
try {
ObjectInputStream ois = new ObjectInputStream(s.getInputStream());
kz = (KlijentskiZahtev) ois.readObject();
} catch (Exception ex) {
Logger.getLogger(ObradaZahtevaNit.class.getName()).log(Level.SEVERE, null, ex);
}
return kz;
}
private void posaljiOdgovor(ServerskiOdgovor so) {
ObjectOutputStream oos;
try {
oos = new ObjectOutputStream(s.getOutputStream());
oos.writeObject(so);
} catch (IOException ex) {
Logger.getLogger(ObradaZahtevaNit.class.getName()).log(Level.SEVERE, null, ex);
}
}
}
<file_sep>• Client-Server application, socket communication
• Application allows logged-in users to manipulate with data about dancer and competition (enter new, delete, edit, search)
| 3597132406930632e366d4e0c6112720805b0a8b | [
"Markdown",
"Java"
] | 4 | Java | vasicnada/PlesniKlub | a51f6c0fb96f3e97617c8eef5b8de235ad6427fe | 0e4084f37a8f9aa9291dc19f74d7b1592bb5803f | |
refs/heads/master | <file_sep>// The MIT License (MIT)
//
// Copyright (c) 2016, 2017 <NAME>
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in
// all copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
// THE SOFTWARE.
#define _GNU_SOURCE
#include <stdio.h>
#include <unistd.h>
#include <sys/wait.h>
#include <stdlib.h>
#include <errno.h>
#include <string.h>
#include <signal.h>
#define WHITESPACE " \t\n" // We want to split our command line up into tokens
// so we need to define what delimits our tokens.
// In this case white space
// will separate the tokens on our command line
#define MAX_COMMAND_SIZE 255 // The maximum command-line size
// MY CODE STARTS HERE
#define MAX_NUM_ARGUMENTS 10 // Mav shell only supports five arguments
#define MAX_HISTORY 15 // Mav shell will only store history of last 10 entries
int status;
struct cmd
{
char *token[MAX_NUM_ARGUMENTS];
pid_t pid;
};
struct cmd history[MAX_HISTORY];
int counter = 0;
int first_null( char *array[MAX_NUM_ARGUMENTS] )
{
int first_null = 0;
for(int i = 0; i < MAX_NUM_ARGUMENTS; i++)
{
if( array[i] == NULL )
{
break;
}
first_null++;
}
return first_null;
}
char * concat_str( char * str1, char * str2 )
{
// +1 is added for null space
char *final = malloc( strlen(str1) + strlen(str2) + 1);
strcpy( final, str1 );
strcat( final, str2 );
return final;
}
static void handle_signal ( int sig )
{
/*
Determine which of the two signals were caught and
print an appropriate message.
*/
switch( sig )
{
pid_t pid;
case SIGINT:
//printf("Caught a SIGINT\n");
break;
case SIGTSTP:
//printf("Caught a SIGTSTP\n");
break;
case SIGCHLD:
pid = waitpid(-1, &status, WNOHANG);
printf("%d\n", pid);
break;
default:
printf("Unable to determine the signal\n");
break;
}
}
// MY CODE ENDS HERE
int main()
{
// MY CODE STARTS HERE
// SET UP SIGNALS
struct sigaction act;
/*
Zero out the sigaction struct
*/
memset (&act, '\0', sizeof(act));
/*
Set the handler to use the function handle_signal()
*/
act.sa_handler = &handle_signal;
/*
Install the handler for SIGINT and SIGTSTP and check the
return value.
*/
if (sigaction(SIGINT , &act, NULL) < 0)
{
perror ("sigaction: ");
return 1;
}
if (sigaction(SIGTSTP , &act, NULL) < 0)
{
perror ("sigaction: ");
return 1;
}
if (sigaction(SIGCHLD , &act, NULL) < 0)
{
perror ("sigaction: ");
return 1;
}
// MY CODE ENDS HERE
char * cmd_str = (char*) malloc( MAX_COMMAND_SIZE );
while( 1 )
{
// Print out the msh prompt
printf ("msh> ");
// Read the command from the commandline. The
// maximum command that will be read is MAX_COMMAND_SIZE
// This while command will wait here until the user
// inputs something since fgets returns NULL when there
// is no input
while( !fgets (cmd_str, MAX_COMMAND_SIZE, stdin) );
/* Parse input */
char *token[MAX_NUM_ARGUMENTS];
int token_count = 0;
// Pointer to point to the token
// parsed by strsep
char *arg_ptr;
char *working_str = strdup( cmd_str );
// we are going to move the working_str pointer so
// keep track of its original value so we can deallocate
// the correct amount at the end
char *working_root = working_str;
// Tokenize the input stringswith whitespace used as the delimiter
while ( ( (arg_ptr = strsep(&working_str, WHITESPACE ) ) != NULL) &&
(token_count<MAX_NUM_ARGUMENTS))
{
token[token_count] = strndup( arg_ptr, MAX_COMMAND_SIZE );
if( strlen( token[token_count] ) == 0 )
{
token[token_count] = NULL;
}
token_count++;
}
// Now print the tokenized input as a debug check
// MY CODE STARTS HERE
// if there are token then the program should not try to change
// directories or execute any program
if( first_null(token) != 0 )
{
// check whether the inputed string is cd, because if
// the command is cd we do not need fork.
if( strcmp(token[0], "cd" ) == 0 )
{
chdir( token[1] );
}
else if ( strcmp(token[0], "bg" ) == 0 )
{
int status;
pid_t changed = waitpid(0, &status, WNOHANG);
printf("%d\n",changed);
}
else if( (strcmp(token[0], "exit") == 0 ) || (strcmp(token[0], "quit") == 0 ) )
{
printf("exit\n");
return 0;
}
// for every other command we need to call, we need to fork
// this is because it is an independed process that we are
// are calling
else
{
pid_t pid = fork();
if( pid == -1 )
{
// When fork() returns -1, an error happened.
perror("fork failed: ");
}
// When fork() returns 0, we are in the child process.
// in child we exec the user input
else if ( pid == 0 )
{
// record so we can kill or get history
// start process in order of folders
char *path = concat_str( "./", token[0] );
ssize_t err = execv( path, token );
// check if process is sucessfully running. If not
// look for executable in next directory
if ( err < 0 )
{
char *path = concat_str( "/usr/local/bin/", token[0] );
ssize_t err = execv( path, token );
// check if process is sucessfully running. If not
// look for executable in next directory
if ( err < 0 )
{
char *path = concat_str( "/usr/bin/", token[0] );
ssize_t err = execv( path, token );
if ( err < 0 )
{
char *path = concat_str( "/bin/", token[0] );
ssize_t err = execv( path, token );
if ( err < 0 )
{
printf("%s: Command not found.\n\n", token[0]);
kill(getpid(),6);
}
}
}
}
free( path );
fflush(NULL);
}
else
{
// Force the parent process to wait until the child process
// exits
pause();
//printf("Hello from the parent process\n\tPID: %d\n\tPPID: %d\n", getpid(),getppid());
fflush( NULL );
}
}
}
// MY CODE ENDS HERE
int token_index = 0;
/*for( token_index = 0; token_index < token_count; token_index ++ )
{
printf("token[%d] = %s\n", token_index, token[token_index] );
}*/
free( working_root );
}
return 0;
}
<file_sep>mfs: mfs.c loop.c
gcc -o msh mfs.c
gcc -o loop loop.c
clean:
rm msh | f5e6f77e6f7246f57e12743f4f8acc74fa2e7183 | [
"C",
"Makefile"
] | 2 | C | VijitSingh97/Maverick-Shell | b6d5bb5d0e367fddbf8e2917ffa918b8462688b9 | 54954ca4f24ef10ec1597fa92894dad75800884b | |
refs/heads/main | <file_sep>import React from 'react';
import styled from 'styled-components';
import { Section } from '../components';
import { THEME } from '../config';
import GeneralLayout from '../layouts/general';
import '../styles/index.css';
/**
尺寸定义全部使用 EM
相对于 BlogContentArea 设置的 FontSize
*/
// https://github.com/markdowncss/retro/blob/master/css/retro.css
const BlogContentArea = styled.div`
max-width: ${THEME.size.sectionContainerWidthMax};
margin: 0 auto;
padding: 1em;
font-family: "Courier New";
font-size: 16px;
line-height: 1.45em;
display: flex;
justify-content: center;
@media screen and (max-width: 1300px) {
flex-direction: column-reverse;
font-size: 10px;
line-height: 2.6em;
}
`;
const MarkdownContent = styled.div`
flex-grow: 1;
max-width: 100%;
padding: 0 1em;
min-height: 100%;
& code {
color: #111;
background: #ddd;
}
& pre {
margin: .5em 0;
padding: .5em;
overflow-x: scroll;
background-color: #333;
}
& pre code {
max-width: 100%;
font-family: Menlo, Monaco, "Courier New", monospace;
color: #fff;
background: transparent;
font-size: .6em;
}
& a, a:visited {
color: #0000ff;
}
& a:hover, a:focus, a:active {
color: #007cff;
}
& ul ul {
padding: 0 1.6em;
/* list-style-type: disc; */
/* list-style-type: circle; */
}
& ul ul ul {
list-style-type: square;
}
&.retro-no-decoration {
text-decoration: none;
}
& p, .retro-p {
margin-bottom: .6em;
}
& h1, .retro-h1, h2, .retro-h2, h3, .retro-h3, h4, .retro-h4 {
margin: 1.414em 0 .5em;
}
& h1, .retro-h1 {
width: 100%;
text-align: center;
margin-top: 0;
font-size: 1.8em;
letter-spacing: .16em;
}
& h2, .retro-h2 {
font-size: 1.6em;
}
& h3, .retro-h3 {
font-size: 1.2em;
}
& h4, .retro-h4 {
font-size: 1em;
}
& h5, .retro-h5 {
font-size: .8em;
}
& h6, .retro-h6 {
font-size: .7em;
}
& small, .retro-small {
font-size: .6em;
}
& blockquote {
margin: 1em 0;
padding-left: 1em;
border-left: .6em solid ${THEME.color.primary.border};
}
& img, canvas, iframe, video, svg, select, textarea {
max-width: 100%;
}
& table {
border-collapse: collapse;
border-spacing: 0;
empty-cells: show;
border: 1px solid #cbcbcb;
}
& thead {
background-color: #666666;
color: #fff;
/* text-align: left; */
vertical-align: bottom;
letter-spacing: .2em;
}
& td,th {
margin: 0;
padding: .5em 1em;
border-left: 1px solid #cbcbcb;
border-width: 0 0 0 1px;
font-size: inherit;
overflow: visible;
}
& tr:nth-child(2n+1) {
background-color: transparent;
}
& tr:nth-child(2n+0) {
background-color: #f9f9f9;
}
& caption {
color: #000;
font: italic 85%/1 arial,sans-serif;
padding: 1em 0;
text-align: center;
}
`;
const SideColumn = styled.div`
padding: 0 1em;
@media screen and (max-width: 1024px) {
padding: 2em 1em;
}
`;
const Tag = styled.span`
margin-left: 1em;
color: #999;
text-decoration: underline;
`;
const TagsList = styled.p`
width: 100%;
margin-top: .2em;
display: flex;
flex-wrap: wrap;
& span {
/* color: #999; */
font-weight: bold;
letter-spacing: .16em;
}
`;
const Details = styled.div`
width: 100%;
/* border: solid 1px red; */
& h5, h6, p {
display: inline-block;
line-height: 2em;
font-size: .8em;
}
& h5 {
color: ${THEME.color.primary.ft};
}
& h6 {
font-weight: normal;
}
`;
export default function Blog({pageContext}) {
// console.log(98989898, pageContext)
const at = (pageContext?.frontmatter?.tags?.length > 0) ? pageContext?.frontmatter?.tags?.split(';') : null;
const ak = (pageContext?.frontmatter?.keywords?.length > 0) ? pageContext?.frontmatter?.keywords?.split(';') : null;
const ss = [];
if (!!at && (at.length > 0)) ss.push(...at);
if (!!ak && (ak.length > 0)) ss.push(...ak);
const tags = Array.from(new Set(ss));
const context = pageContext?.frontmatter || {};
return (
<GeneralLayout context={{
...context,
head: pageContext?.frontmatter?.title,
}} >
<Section style={{background: '#fff', padding: '1em'}}>
<BlogContentArea>
<MarkdownContent dangerouslySetInnerHTML={{__html: pageContext?.html}} />
<SideColumn >
<Details>
<table style={{width: '100%', marginBottom: '1.2em'}}>
<tr>
<td><h5>主题:</h5><h6>{`${pageContext?.frontmatter?.subject}`}</h6></td>
<td><h5>类别:</h5><h6>{`${pageContext?.frontmatter?.category}`}</h6></td>
</tr>
<tr>
<td><h5>更新:</h5><h6>{`${pageContext?.frontmatter?.updated_when}`}</h6></td>
<td><h5>创建:</h5><h6>{`${pageContext?.frontmatter?.created_when}`}</h6></td>
</tr>
<tr>
<td><h5>作者:</h5><h6>{`${pageContext?.frontmatter?.author}`}</h6></td>
</tr>
</table>
{ !tags ? null :
<TagsList>
<span>{'标签&关键字:'}</span>
{ tags.map((t) => (
<Tag>{t}</Tag>
)) }
</TagsList>
}
</Details>
</SideColumn >
</BlogContentArea>
</Section>
</GeneralLayout>
)
}
<file_sep>import React from 'react';
import styled from 'styled-components';
import { Link } from 'gatsby';
import { MENU_SETTING } from './menu.setting';
import { SVGTriangle } from './SVG';
/**
Styled Components
*/
const MenuContainer = styled.nav`
width: 100%;
margin: 0;
padding: 0;
box-sizing: border-box;
white-space: nowrap;
font-size: 10px;
height: ${ (props) => (props.height || MENU_SETTING.NAV_MENU_HEIGHT) };
& a {
text-decoration: none;
}
& ul {
list-style: none;
}
@media screen and (max-width: ${MENU_SETTING.SCREEN_WIDTH_THRESHOLD}) {
display: none;
}
`;
const Menu = styled.ul`
width: 100%;
display: flex;
justify-content: space-between;
`;
const SubMenu = styled.ul`
display: block;
position: absolute;
z-index: 999999;
top : ${ (props) => (props.ext ? '.3rem' : '100%')};
left: ${ (props) => (props.ext ? '100%' : '0')};
background: ${ (props) => (` ${props.color?.subBg || 'rgba(255, 255, 255, 0.6)' }`) };
border-top : ${ (props) => (props.ext ? 'none' : `solid 3px ${props.color?.arrow}`)};
border-left : ${ (props) => (props.ext ? `solid 3px ${props.color?.arrow}` : 'none')};
visibility: hidden;
opacity: 0;
transition: all .6s ease;
&:before {
content: '';
position: absolute;
top: ${ (props) => (props.ext ? '.2rem' : '-1.3rem') };
left: ${ (props) => (props.ext ? '-1.3rem' : '1rem') };
border: solid .6rem transparent;
border-bottom-color : ${ (props) => (props.ext ? 'transparent' : (props.color?.arrow)) };
border-right-color : ${ (props) => (props.ext ? (props.color?.arrow) : 'transparent') };
}
`;
const MenuItem = styled.li`
position: relative;
line-height: ${MENU_SETTING.NAV_MENU_HEIGHT};
& a {
display: block;
padding: 0 .5rem;
font-size: 1.1rem;
color: ${(props) => props.color?.ft || '#eee'};
transition: color 0.6s;
}
& a:hover {
color: ${(props) => props.color?.ftHover || '#fff'};
}
&:hover > ${SubMenu} {
visibility: visible;
opacity: 1;
}
`;
const SubMenuItem = styled(MenuItem)`
line-height: 2rem;
`;
/**
Function Components
*/
const NavSub = (props) => {
const {items, ext} = props;
return(
!items ? null :
<SubMenu {...props} ext={ext}>
{ items?.map((item, index) => (
<SubMenuItem key={index} active={index === -1}>
<Link to={item.url} style={{width: '100%', display: 'flex', justifyContent: 'space-between', alignItems: 'center'}}>
<span>{item.text}</span>
{ !item.sub ? null :
<div style={{ height: '100%', marginLeft: '.3em', display: 'inline-flex', justifyContent: 'center', alignItems: 'center'}}>
<SVGTriangle width=".8em" height=".8em" color={props.color.ft} direction="right" />
</div>
}
</Link>
{ !item.sub ? null : <NavSub {...props} ext={true} items={item.sub} /> }
</SubMenuItem>
)) }
</SubMenu>
)
}
export const NavMenu = (props) => {
const { items } = props;
return (
!items ? null :
<MenuContainer {...props}>
<Menu>
{ items?.map((item, index) => (
<MenuItem {...props} key={index} active={index === -1} >
<Link to={item.url} style={{width: '100%', display: 'flex', justifyContent: 'space-between', alignItems: 'center'}}>
<span>
{item.text}
</span>
{ !item.sub ? null :
<div style={{ height: '100%', marginLeft: '.3em', display: 'inline-flex', justifyContent: 'center', alignItems: 'center'}}>
<SVGTriangle width=".8em" height=".8em" color={props.color.ft} />
</div>
}
</Link>
{ !item.sub ? null : <NavSub {...props} items={item.sub} /> }
</MenuItem>
)) }
</Menu>
</MenuContainer>
)
}
<file_sep>// import React from 'react';
import styled from 'styled-components';
import { THEME } from '../config';
export const Button = styled.button`
border-radius: 0;
padding: .5rem 1rem;
outline: none;
white-space: nowrap;
cursor: pointer;
color: ${({ primary }) => (primary ? '#fff' : THEME.color.primary.bg)};;
border: ${({ primary }) => (primary ? 'solid 1px #fff' : `solid 1px ${THEME.color.primary.bg}`)};;
background: ${({ primary }) => (primary ? THEME.color.primary.bg : 'none')};
& svg {
width: 1rem;
height: 1rem;
margin-left: .5rem;
border-radius: 50%;
border: solid 1px;
transform: translateY(.2rem);
}
&:hover {
transition: all .3s ease-out;
background: ${THEME.color.primary.bg};
color: #fff;
}
@media screen and (max-width: 991px) {
width: 100%;
}
`;
<file_sep>import React, { useState } from 'react';
import styled from 'styled-components';
import { Link } from 'gatsby';
import { Bars } from './bars';
import { MENU_SETTING } from './menu.setting';
import { SVGTriangle } from './SVG';
/**
Styled Components
*/
const Menu = styled.ul`
position: relative;
width: 100vw;
height: 100vh;
padding: 6em 2em;
line-height: 3em;
display: block;
z-index: 9999;
font-size: 24px;
background: ${ (props) => ( props.color?.bg) };
transform: ${(props) => !props.open ? `translateX(110vw)` : `translateX(0)` } ;
transition: all .6s ease;
`;
const SubMenu = styled.ul`
margin: 0 0 0 1.2em;
display: none;
visibility: visible;
opacity: 1;
`;
const MenuItem = styled.li`
border-top: solid 1px #999;
& a {
display: block;
padding: 0 .5em;
font-size: 1.1em;
color: ${(props) => props.color?.ft || '#ddd'};
transition: color 0.6s;
}
& a:hover {
color: ${(props) => props.color?.ftHover || '#fff'};
}
&:hover > ${SubMenu} {
display: block;
visibility: visible;
opacity: 1;
}
`;
/**
Function Components
*/
const RecurMenu = (props) => {
const {items, open, ext} = props;
return (
!items ? null :
<SubMenu className='menu' {...props} ext={ext} open={open} >
{ items?.map((item, index) => (
<MenuItem {...props} key={index} active={index === -1}>
<Link to={item.url} style={{width: '100%', display: 'flex', justifyContent: 'space-between', alignItems: 'center'}}>
<span>{item.text}</span>
{ !item.sub ? null :
<div style={{ height: '100%', marginLeft: '.3em', display: 'inline-flex', justifyContent: 'center', alignItems: 'center'}}>
<SVGTriangle width=".8em" height=".8em" color={props.color.ft} />
</div>
}
</Link>
{ !item.sub ? null : <RecurMenu {...props} ext={true} items={item.sub} /> }
</MenuItem>
)) }
</SubMenu>
)
}
const MainMenu = (props) => {
const {items, open, ext} = props;
return (
!items ? null :
<Menu className='menu' {...props} ext={ext} open={open} >
{ items?.map((item, index) => (
<MenuItem {...props} key={index} active={index === -1}>
<Link to={item.url} style={{width: '100%', display: 'flex', justifyContent: 'space-between', alignItems: 'center'}}>
<span>{item.text}</span>
{ !item.sub ? null :
<div style={{ height: '100%', marginLeft: '.3em', display: 'inline-flex', justifyContent: 'center', alignItems: 'center'}}>
<SVGTriangle width=".8em" height=".8em" color={props.color.ft} />
</div>
}
</Link>
{ !item.sub ? null : <RecurMenu {...props} ext={true} items={item.sub} /> }
</MenuItem>
)) }
</Menu>
)
}
const MenuContainer = styled.div`
position: fixed;
height: 1px; // 防止这一层会遮盖住 blog list
font-size: 10px;
& a {
text-decoration: none;
}
& ul {
list-style-type: none;
}
@media screen and (min-width: ${MENU_SETTING.SCREEN_WIDTH_THRESHOLD}) {
display: none;
}
`;
const PopButton = styled.div`
cursor: pointer;
position: absolute;
top: 1em;
right: 2em;
z-index: 999999;
`;
export const PopMenu = (props) => {
const {items, color} = props;
const [open, setOpen] = useState(false);
return (
<MenuContainer className='container' {...props} >
<PopButton className='button' onClick={() => setOpen(!open)} >
<Bars open={open} color={color.ft} ></Bars>
</PopButton>
<MainMenu {...props} open={open} items={items} />
</MenuContainer>
)
}
<file_sep>import React from "react";
import { graphql, useStaticQuery } from 'gatsby';
import styled from 'styled-components';
import { THEME } from '../config';
import { Section, CenterColumn, NavMenu, PopMenu } from '../components';
const JumbotronArea = styled.div`
width: 100%;
padding: 2em 0;
font-size: 16px;
line-height: 2em;
display: flex;
justify-content: center;
align-items: center;
& img {
width: 30%;
}
@media screen and (max-width: 800px) {
font-size: 12px;
flex-direction: column-reverse;
& img {
width: 50%;
}
}
@media screen and (max-width: 450px) {
font-size: 10px;
}
`;
const Slogan = styled.div`
width: 50%;
height: 100%;
margin: 0 2em;
display: flex;
flex-direction: column;
color: #e0e0e0;
& h1 {
line-height: 2.3em;
font-size: 2.8em;
}
& p {
font-size: 1.2em;
line-height: 1.6em;
letter-spacing: .1em;
}
@media screen and (max-width: 800px) {
width: 80%;
}
`;
export default function JumbotronSection() {
const data = useStaticQuery(graphql`
query {
site {
siteMetadata {
title
author
description
jumbotron
navItems {
key
url
text
sub {
key
url
text
sub {
key
url
text
}
}
}
}
}
}
`);
return (
<Section style={{background: `linear-gradient(90deg,${THEME.color.primary.border},${THEME.color.primary.ft})` }}>
<CenterColumn>
<PopMenu color={{ ft: '#eee', bg: THEME.color.primary.border }} items={data.site.siteMetadata.navItems} />
<div style={{width: '100%', display: 'flex', justifyContent: 'center'}}>
<div style={{width: '20rem', color: '#fff'}} >
<NavMenu items={data.site.siteMetadata.navItems} color={{
subBg: THEME.color.primary.border,
arrow: '#fff',
ft: '#fff',
}}/>
</div>
</div>
<JumbotronArea>
<Slogan>
<h1>{data.site.siteMetadata.title}</h1>
<p>{data.site.siteMetadata.description}</p>
</Slogan>
<img src={data.site.siteMetadata.jumbotron} alt='coding' />
</JumbotronArea>
</CenterColumn>
</Section>
)
}
<file_sep>import styled from 'styled-components';
export const Bars = styled.span`
width: 2em;
height: .2em;
background: ${(props) => (!!props.open ? 'transparent' : (props.color || '#999'))} ;
display: inline-block;
position: relative;
transition: all .2s ease;
&:before,&:after {
content: '';
width: 2em;
height: .2em;
background: ${(props) => (props.color || '#999')} ;
display: inline-block;
position: absolute;
}
&:before {
top : ${(props) => (!props.open ? '.5em' : '0')};
transform : ${(props) => (!props.open ? 'none' : 'rotate(45deg)')};
}
&:after {
top : ${(props) => (!props.open ? '-.5em' : '0')};
transform : ${(props) => (!props.open ? 'none' : 'rotate(135deg)')};
}
`;
<file_sep>export const MENU_SETTING = {
SCREEN_WIDTH_THRESHOLD: '576px',
NAV_MENU_HEIGHT: '4em',
}
<file_sep>export * from './elements';
export * from './bars';
export * from './search';
export * from './Head';
export * from './Button';
export * from './Menu';
export * from './Menu.Pop';
export * from './ShortCutToTop';
export * from './Slider';
<file_sep>import React from 'react';
export const SVGTriangle = ({width, height, direction, color}) => (
<svg xmlns="http://www.w3.org/2000/svg" width={width} height={height} viewBox="0 0 16 16">
<polygon points={ direction === 'right' ? "4,3 13,9 4,14" : "2,4 14,4 9,13"} style={{
fill: color,
}} />
</svg>
)
<file_sep>import React from "react";
import HomeLayout from '../layouts/home';
import '../styles/index.css';
export default function HomePage() {
return (
<HomeLayout context={{ head: '首页' }} />
)
}
<file_sep># blog
This is a fundamental web site source code repo.
This project is mainly based on React & Gatsby.<file_sep>import React from "react"
import { Link } from 'gatsby';
import '../styles/index.css';
export default function NotFound() {
return (
<>
<h1>Page not found!</h1>
<Link to="/">Back to Home</Link>
</>
)
}
<file_sep>import React from 'react';
import { Head, PageTopAnchor, ShortCutToTop } from '../components';
import JumbotronSection from './section.jumbotron';
import BlogListSection from './section.bloglist';
import FooterSection from './section.footer';
export default function HomeLayout ({context}) {
return (
<>
<Head context={context} />
<PageTopAnchor />
<ShortCutToTop>👍</ShortCutToTop>
<JumbotronSection />
<BlogListSection />
<FooterSection />
</>
)
}
<file_sep>import React, { useState, useRef, useMemo, useEffect } from 'react';
import styled, { keyframes, css } from 'styled-components';
// import { THEME } from '../config';
const ANIMATION_TOTAL = 100; // 动画整体进度为 100%
const move2Left = (width, timings) => keyframes`
0% { margin-left: 0; }
${timings.map((times, index) => (
`${times[0]}%, ${times[1]}% { margin-left: -${width * index}px; }`
))}
/* 100% { margin-left: 0; } */
`;
const SliderContainer = styled.div`
position: relative;
width : ${ (props) => `${props?.size?.width}px` };
height: ${ (props) => `${props?.size?.height}px` };
/* border: solid 5px #f00; */
`;
const SliderWindow = styled.div`
/* border: solid 5px #0f0; */
position: absolute;
top: 0;
left: 0;
width : ${ (props) => `${props?.size?.width}px` };
height: ${ (props) => `${props?.size?.height}px` };
overflow: hidden;
`;
// props.focusId 只有在 animation 没有的情况下,才有效果
const SliderGallery = styled.div`
position: absolute;
top: 0;
left: 0;
margin-left: ${(props) => `-${ (props.focusId || 0) * props?.width }px`};
display: flex;
animation: ${ (props) => (props.focusId !== null) ? '' : css`${move2Left(props?.width, props?.timings)} ${props.duration}s linear infinite` };
`;
export const SliderItem = styled.div`
/* border: solid 1px #ff0000; */
position: relative;
width : ${ (props) => `${props?.size?.width}px` };
height: ${ (props) => `${props?.size?.height}px` };
& img {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
}
& p {
position: absolute;
bottom: 0;
left: 0;
width: 100%;
background: rgba(0, 0, 0, 0.6);
color: #fff;
text-align: center;
overflow: hidden;
}
`;
const SliderItemIndicator = styled.div`
position: absolute;
bottom: 0;
left: 0;
width: 100%;
display: flex;
justify-content: center;
`;
const SliderIndicatorDot = styled.button`
width: 16px;
height: 16px;
border: solid 3px #333;
border-radius: 50%;
margin: 2rem .2rem;
background: ${ (props) => ( props.active ? 'yellow' : 'grey' ) };
`;
export const Slider = ({size, duration, children}) => {
const [focusId, setFocusId] = useState(null);
const [, setTrigger] = useState(0);
const timerRef = useRef(null);
const galleryRef = useRef();
useEffect(() => {
console.log('effect')
timerRef.current = setInterval(() => {
// console.log('interval', trigger); // !!! 这里的 trigger 始终都不会改变,似乎被 “冻住” 了 (无法访问外部的变量,而外部的变量其实是变化了的) !!!
setTrigger(Math.random()); // 所以,这里设置随机数,来触发界面刷新
}, 1000);
return () => {
console.log('Bye', timerRef.current);
clearInterval(timerRef.current);
}
}, []);
const timings = useMemo(() => {
const length = children?.length || 1;
const trans = ANIMATION_TOTAL / length / 10; // 图片与图片间的过场动画时间
const result = children.map((x, index) => ([trans, ANIMATION_TOTAL * (index + 1) / length]));
result.forEach((timing, index) => {
if (index > 0) timing[0] = result[index - 1][1] + trans;
});
return result;
}, [children]);
const styleMarginLeft = !galleryRef?.current ? 0 : window.getComputedStyle(galleryRef.current).getPropertyValue("margin-left");
const currentImgId = (focusId !== null) ? focusId : (-parseInt(parseInt(styleMarginLeft)/size.width));
// console.log(galleryRef, styleMarginLeft);
// console.log(trigger, currentImgId);
return (
!children?.length ? null :
// <>
// <h1>{trigger}</h1>
// <button onClick={() => setTrigger(trigger +1)}>+</button>
// </>
<SliderContainer size={size}>
<SliderWindow size={size}>
<SliderGallery ref={galleryRef} width={size.width} timings={timings} duration={duration * (children?.length || 0)} focusId={focusId}>
{ children.map((child, index) => {
const newChild = React.cloneElement(child, {
...child?.props,
size: size,
});
return newChild;
}) }
</SliderGallery>
<SliderItemIndicator>
{ children.map((child, index) => ( <SliderIndicatorDot active={index === currentImgId} onClick={ () => setFocusId( (focusId !== index) ? index : null) }></SliderIndicatorDot> ))}
</SliderItemIndicator>
</SliderWindow>
</SliderContainer>
)
}
<file_sep>import React from "react";
import styled from 'styled-components';
import { graphql, useStaticQuery } from 'gatsby';
import { THEME } from '../config';
import { Section } from '../components';
const FooterArea = styled.div`
max-width: ${THEME.size.sectionContainerWidthMax};
min-height: 6em;
margin: 0 auto;
padding: 1em;
font-size: 16px;
display: flex;
justify-content: space-between;
@media screen and (max-width: 800px) {
line-height: 2em;
font-size: 10px;
flex-direction: column-reverse;
align-items: center;
}
`;
export default function FooterSection() {
const data = useStaticQuery(graphql`
query {
site {
siteMetadata {
copyright
contact {
phone
email
}
}
}
}
`);
return (
<Section style={{ background: THEME.color.dark.bg, color: '#fff' }}>
<FooterArea>
<span>{data?.site?.siteMetadata?.copyright}</span>
<div>
<span role="img" aria-label="Phone" >☎️</span>
<span>{data?.site?.siteMetadata?.contact?.phone}</span>
<span style={{margin: '0 .7em'}}></span>
<span role="img" aria-label="Email" >📧</span>
<span>{data?.site?.siteMetadata?.contact?.email}</span>
</div>
<span role="img" aria-label="Goodness" >✨ 🍺 🏠 ☕ 🌈 🇨🇳 </span>
</FooterArea>
</Section>
)
}
<file_sep>import React from 'react';
export const PageTopAnchor = () => (<div id='__page_top__' />)
export const ShortCutToTop = ({children}) => (
<div style={{
position: 'fixed',
bottom: '2rem',
right: '2rem',
zIndex: '99999',
fontSize: '2rem',
}} >
<a href='#__page_top__'><span role="img" aria-label="Up">{children}</span></a>
</div>
)
<file_sep>export const THEME = {
size: {
sectionContainerWidthMax: '1300px',
},
// https://colorbrewer2.org/#type=sequential&scheme=PuBuGn&n=3
color: {
primary : { bg: '#00A6CB', border: '#00818e', ft: '#00b8cb' },
secondary : { bg: '#e4fd63', border: '#e4fd63', ft: '#e4fd63' },
normal : { bg: '#e6e6e6', border: '#adadad', ft: '#333', },
// primary : { bg: '#286090', border: '#204d74', },
success : { bg: '#cb008b', border: '#8e0061', },
info : { bg: '#bff9ff', border: '#80f3ff', },
warning : { bg: '#cba600', border: '#8e7400', },
danger : { bg: '#cb5500', border: '#8e3c00', },
link : { ft: '#23527c'},
dark : { bg: '#262626'},
},
}
/**
https://www.shutterstock.com/zh/color/cyan-blue
Single : #00A6CB, #00b8cb, #00818e, #bff9ff, #80f3ff
Similar : #052fcb, #04218e
Contrast: #cb5500, #8e3c00
Triangle: #cb008b, #8e0061, #cba600, #8e7400
*/
/**
https://www.shutterstock.com/zh/color/teal-blue
Single : #00807E, #008080, #005a5a, #bfffff, #80ffff
Similar : #002080, #00165a
Contrast: #803300, #5a2400
Triangle: #800060, #5a0043, #806600, #5a4800
*/
/**
https://www.shutterstock.com/zh/color/bottle-green
Single : #006a4e, #006a5a, #004a3f, #bffff5, #80ffeb
Similar : #00256a, #001a4a
Contrast: #6a2300, #4a1900
Triangle: #6a0057, #4a003d, #6a5100, #4a3900
*/
<file_sep>---
title: markdown
subtitle: markdown
subject: subject
category: category
tags: tag
keywords: kw
level: 100
cover: http://qiniuargus.weready.online/blog/coder.jpg
author: <NAME>
created_when: 2021-12-31
updated_when: 2021-12-31
---
# debugging
- step 1
- step 2
+ step 2.1
+ step 2.2
* step 2.2.1
<file_sep>import React, { useState } from "react";
import styled from 'styled-components';
import { Head, NavMenu, PopMenu } from '../components';
import '../styles/index.css';
const OPTIONS = [
{ bg: '#0062be', img: "http://qiniuargus.weready.online/storybooks/pepsi/pepsi001.png"},
{ bg: '#e60c2c', img: "http://qiniuargus.weready.online/storybooks/pepsi/pepsi002.png"},
{ bg: '#1e1e1e', img: "http://qiniuargus.weready.online/storybooks/pepsi/pepsi003.png"},
];
const SETTING = {
distanceSide: '6em',
}
const Section = styled.section`
position: relative;
min-height: 100vh;
font-size: 16px;
display: flex;
flex-direction: column;
justify-content: space-between;
@media screen and (max-width: 500px) {
font-size: 10px;
}
`;
const HeaderBar = styled.div`
padding: 1em ${SETTING.distanceSide};
display: flex;
justify-content: space-between;
align-items: center;
`;
const Jumbotron = styled.div`
position: relative;
margin: 0 ${SETTING.distanceSide};
display: flex;
justify-content: space-between;
align-items: center;
@media screen and (max-width: 500px) {
width: 100%;
flex-direction: column-reverse;
margin: .6em 0;
}
`;
const Selector = styled.ul`
list-style-type: none;
display: flex;
justify-content: center;
& li {
margin: 1em;
transition: .5s;
}
& li:hover {
transform: translateY(-20%);
}
& img {
height: 6em;
}
`;
const Logo = styled.div`
& img {
width: 5em;
height: 5em;
}
`;
const Menu = styled.ul`
`;
const TextArea = styled.div`
width: 50%;
color: #fff;
& h1, h2 {
text-transform: uppercase;
}
& h2 {
font-size: 3.6em;
line-height: 1em;
}
& h1 {
font-size: 7.2em;
line-height: 1em;
}
& p {
margin: 0;
letter-spacing: .06em;
line-height: 1.4em;
text-align: justify;
text-justify: inter-ideograph;/*IE*/
}
@media screen and (max-width: 500px) {
width: 100%;
padding: 0 3.6em;
}
`;
const Image = styled.div`
width: 50%;
display: flex;
justify-content: center;
align-items: center;
@media screen and (max-width: 500px) {
width: 100%;
margin: 3.2em;
}
`;
const Contact = styled.ul`
position: absolute;
right: 1rem;
top: 50%;
transform: translateY(-50%); */
list-style: none;
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
& li {
padding: 1em 0;
list-style: none;
cursor: pointer;
transition: .5s;
}
& li:hover {
transform: scale(1.1);
}
& img {
filter: invert(1);
}
@media screen and (max-width: 500px) {
display: none;
}
`;
const navItems = [
{ key: 'home', url: '/', text: 'Home' },
{ key: 'products', url: '/#', text: 'Products' },
{ key: 'whatisnew', url: '/#', text: "What's New" },
{ key: 'newsletter',url: '/#', text: 'Newsletter' },
{ key: 'contact', url: '/#', text: 'Contact' },
];
export default function LandingPage() {
const [index, setIndex] = useState(0);
return (
<>
<Head context={{ head: '百事可乐' }} />
<Section style={{ background: OPTIONS[index].bg }}>
<HeaderBar>
<Logo>
<img src="http://qiniuargus.weready.online/storybooks/pepsi/logo.png" />
</Logo>
<Menu>
<NavMenu items={navItems} color={{
subBg: OPTIONS[index].bg,
arrow: '#fff',
}}/>
</Menu>
</HeaderBar>
<Jumbotron className="jumbotron">
<TextArea>
<h2>That's what</h2>
<h1>I like</h1>
<p>It is not the critic who counts; not the man who points out how the strong man stumbles, or where the doer of deeds could have done them better. The credit belongs to the man who is actually in the arena, whose face is marred by dust and sweat and blood; who strives valiantly; who errs, who comes short again and again, because there is no effort without error and shortcoming; but who does actually strive to do the deeds; who knows great enthusiasms, the great devotions; who spends himself in a worthy cause; who at the best knows in the end the triumph of high achievement, and who at the worst, if he fails, at least fails while daring greatly, so that his place shall never be with those cold and timid souls who neither know victory nor defeat.</p>
</TextArea>
<Image>
<img src={OPTIONS[index].img} />
</Image>
</Jumbotron>
<Selector>
{ OPTIONS.map((op, id) => (
<li onClick={() => setIndex(id)}><img src={op.img} /></li>
))}
</Selector>
<Contact>
<li><img src='http://qiniuargus.weready.online/icons/facebook.png' /></li>
<li><img src='http://qiniuargus.weready.online/icons/instagram.png'/></li>
<li><img src='http://qiniuargus.weready.online/icons/twitter.png' /></li>
</Contact>
<PopMenu color={{ ft: '#999', bg: OPTIONS[index].bg }} items={navItems} />
</Section>
</>
)
}
<file_sep>import React from 'react';
import { Helmet } from 'react-helmet';
import { useStaticQuery, graphql } from 'gatsby';
export const Head = ({context}) => {
const data = useStaticQuery(graphql`
query {
site {
siteMetadata {
title
}
}
}
`);
// console.log(999999, data)
// console.log(999999, data.site.siteMetadata.title)
return(
<Helmet >
<title>
{
(!context || !context.head) ? data.site.siteMetadata.title :
`${data.site.siteMetadata.title} | ${context.head}`
}
</title>
{ !context?.keywords ? null : <meta name='keywords' content={context.keywords} /> }
{ !context?.category ? null : <meta property='wr:type' content={context.category} /> }
{ !context?.title ? null : <meta property='wr:title' content={context.title} /> }
{ !context?.subtitle ? null : <meta property='wr:description' content={context.subtitle} /> }
{/* { !context?.? null : <meta property='wr:image' content={context.} /> }
{ !context?.? null : <meta property='wr:locale' content={context.} /> }
{ !context?.? null : <meta property='wr:url' content={context.} /> } */}
{/* <link rel='canonical' content='' /> */}
}
</Helmet>
)
}
<file_sep>import styled from 'styled-components';
import { THEME } from '../config';
export const Section = styled.div`
width: 100%;
`;
export const Column = styled.div`
height: 100%;
`;
export const CenterColumn = styled(Column)`
margin: 0 auto;
max-width: ${THEME.size.sectionContainerWidthMax};
`;
<file_sep>import React from "react";
import { graphql, useStaticQuery } from 'gatsby';
import { THEME } from '../config';
import { Section, CenterColumn, NavMenu, PopMenu } from '../components';
export default function HeaderSection() {
const data = useStaticQuery(graphql`
query {
site {
siteMetadata {
navItems {
key
url
text
sub {
key
url
text
sub {
key
url
text
}
}
}
}
}
}
`);
return (
<Section style={{background: `linear-gradient(90deg,${THEME.color.primary.border},${THEME.color.primary.ft})` }}>
<CenterColumn>
<PopMenu color={{ ft: '#999', bg: THEME.color.primary.border }} items={data.site.siteMetadata.navItems} />
<div style={{width: '100%', display: 'flex', justifyContent: 'center'}}>
<div style={{width: '20rem', color: '#fff'}} >
<NavMenu items={data.site.siteMetadata.navItems} color={{
subBg: THEME.color.primary.border,
arrow: '#fff',
}}/>
</div>
</div>
</CenterColumn>
</Section>
)
}
| 537b5ad4ee5cd0821e0876559918f643aef0924b | [
"JavaScript",
"Markdown"
] | 22 | JavaScript | we-ready/blog | f750ca0e871d52149ea4eb939fdbaae2bf29f3d0 | 494ef55a0c94c94451e807599f731e7a024e7672 | |
refs/heads/master | <file_sep>import pandas as pd
import numpy as np
from numpy import inf
from rdkit import Chem
from rdkit.Chem.Draw import IPythonConsole
import PYTHON.chemutils as cu
import PYTHON.mol_tree as mt
import PYTHON.chemfun as cf
from rdkit.Chem import Draw
from rdkit.Chem import AllChem
from rdkit.Chem import Descriptors
from mendeleev import element
import h5py
import collections
import keras
from keras.models import Sequential
from keras.layers import Dense,Input
from sklearn.model_selection import StratifiedKFold
from keras.wrappers.scikit_learn import KerasRegressor
import numpy
from matplotlib import pyplot
from sklearn import preprocessing
from sklearn.preprocessing import StandardScaler
from sklearn.preprocessing import MinMaxScaler
from keras.wrappers.scikit_learn import KerasRegressor
from sklearn.model_selection import KFold
from sklearn.model_selection import cross_val_score
from sklearn.metrics import mean_squared_error as mse
from sklearn.metrics import mean_absolute_error as mae
from sklearn.model_selection import RepeatedKFold
from sklearn.ensemble import RandomForestRegressor
from scipy.stats import pearsonr
import matplotlib.pyplot as plt
import matplotlib
import seaborn as sns
import pickle
import GPy
import tensorflow as tf
#from tensorflow import keras
#from tensorflow.keras import layers
from PYTHON.wacsfs import histWACSF, makeDF
from PYTHON.msde_functions import *
from PYTHON.std import *
import warnings
warnings.filterwarnings("ignore")
import os
os.environ['TF_CPP_MIN_LOG_LEVEL'] = '1'
import itertools
<file_sep># MSDE Functions
import keras
from keras.layers import Dense, Input
import PYTHON.chemfun as cf
#import PYTHON.chemfun_1 as cf1
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
import seaborn as sns
from sklearn.metrics import mean_squared_error as mse
from sklearn.metrics import mean_absolute_error as mae
from scipy.stats import pearsonr
from rdkit import Chem
from sklearn.ensemble import RandomForestRegressor
import datetime
# Tensorflow graph being a nuisance and makes optimisation process slow down as the number of models increases,
# trying to make function that resets graphs after each model training
def ResetGraph():
keras.backend.clear_session()
# Building the model #
def build_model(input,architecture, activation, optimiser):
ResetGraph()
input1 = Input(shape = (len(input.columns),))
a = input1
for layer in architecture:
a = Dense(layer,activation = activation)(a)
output1 = Dense(1,activation = 'linear')(a)
model = keras.models.Model(inputs = input1, outputs = output1)
model.compile(optimizer = optimiser, loss='mean_squared_error', metrics=['mean_absolute_error', 'mean_squared_error'])
return model
def GridSearchCVKeras(xtrain_list, xtest_list, ytrain_list, ytest_list, param_grid):
results = {'epochs':[],
'architecture':[],
'activation':[],
'optimiser':[]}
for x in range(1,len(xtrain_list)+1,1):
results['train_mae_hist_%s'%(x)] = []
results['train_mse_hist_%s'%(x)] = []
results['test_mae_hist_%s'%(x)] = []
results['test_mse_hist_%s'%(x)] = []
num_models = 1
for key in param_grid.keys():
num_models*=len(param_grid[key])
counter = 1
for architecture in param_grid['architecture']: # Grid Search
for activation in param_grid['activation']:
for optimiser in param_grid['optimiser']:
for epoch in param_grid['epochs']:
results['architecture'].append(architecture)
results['activation'].append(activation)
results['optimiser'].append(optimiser)
results['epochs'].append([x for x in range(1,epoch+1,1)])
print('Model Number:',counter,' | Current time:', datetime.datetime.now())
index=1
for xtr, xte, ytr, yte in zip(xtrain_list, xtest_list, ytrain_list, ytest_list):
model = build_model(xtr, architecture, activation, optimiser)
history = model.fit(xtr,ytr, validation_data = [xte,yte],epochs = epoch, verbose = 0)
hist = history.history
results['train_mse_hist_%s'%(index)].append(hist['mean_squared_error'])
results['train_mae_hist_%s'%(index)].append(hist['mean_absolute_error'])
results['test_mse_hist_%s'%(index)].append(hist['val_mean_squared_error'])
results['test_mae_hist_%s'%(index)].append(hist['val_mean_absolute_error'])
ResetGraph()
index += 1
counter+=1
return pd.DataFrame(results)
def get_standard_descriptors(mol_smiles, output = None, output_name = 'output'):
mol_rdkit = cf.SMILES2MOLES(mol_smiles) # Converting smiles to RD-Kit format
mol_rdkit_H = cf1.ADDH_MOLES(mol_rdkit) # Adding hydrogens to molecules
descriptors = []
for m in mol_rdkit_H:
desc = []
desc.append(Chem.GraphDescriptors.BalabanJ(m)) # Chem. Phys. Lett. 89:399-404 (1982)
desc.append(Chem.GraphDescriptors.BertzCT(m)) # J. Am. Chem. Soc. 103:3599-601 (1981)
desc.append(Chem.GraphDescriptors.Ipc(m)) # J. Chem. Phys. 67:4517-33 (1977) # Gives very small numbers #
desc.append(Chem.GraphDescriptors.HallKierAlpha(m)) # Rev. Comput. Chem. 2:367-422 (1991)
desc.append(Chem.Crippen.MolLogP(m)) # Wildman and Crippen JCICS 39:868-73 (1999)
desc.append(Chem.Crippen.MolMR(m)) # Wildman and Crippen JCICS 39:868-73 (1999)
desc.append(Chem.Descriptors.ExactMolWt(m))
desc.append(Chem.Descriptors.FpDensityMorgan1(m))
#desc.append(Chem.Descriptors.MaxPartialCharge(m)) # Returns null value for a molecule in lipophilicity dataset #
desc.append(Chem.Descriptors.NumRadicalElectrons(m))
desc.append(Chem.Descriptors.NumValenceElectrons(m))
desc.append(Chem.Lipinski.FractionCSP3(m))
desc.append(Chem.Lipinski.HeavyAtomCount(m))
desc.append(Chem.Lipinski.NumHAcceptors(m))
desc.append(Chem.Lipinski.NumAromaticRings(m))
desc.append(Chem.Lipinski.NHOHCount(m))
desc.append(Chem.Lipinski.NOCount(m))
desc.append(Chem.Lipinski.NumAliphaticCarbocycles(m))
desc.append(Chem.Lipinski.NumAliphaticHeterocycles(m))
desc.append(Chem.Lipinski.NumAliphaticRings(m))
desc.append(Chem.Lipinski.NumAromaticCarbocycles(m))
desc.append(Chem.Lipinski.NumAromaticHeterocycles(m))
desc.append(Chem.Lipinski.NumHDonors(m))
desc.append(Chem.Lipinski.NumHeteroatoms(m))
desc.append(Chem.Lipinski.NumRotatableBonds(m))
desc.append(Chem.Lipinski.NumSaturatedCarbocycles(m))
desc.append(Chem.Lipinski.NumSaturatedHeterocycles(m))
desc.append(Chem.Lipinski.NumSaturatedRings(m))
desc.append(Chem.Lipinski.RingCount(m))
desc.append(Chem.rdMolDescriptors.CalcTPSA(m))
desc.append(Chem.rdMolDescriptors.CalcTPSA(m)) # J. Med. Chem. 43:3714-7, (2000)
desc.append(Chem.MolSurf.LabuteASA(m)) # J. Mol. Graph. Mod. 18:464-77 (2000)
descriptors.append(desc)
descriptors_df = pd.DataFrame(data = descriptors, columns = [x for x in range(len(descriptors[0]))])
if (outputs != None):
descriptors_df[output_name] = output
return descriptors_df
# Decomposes a list of molecules in smiles into cliques and returns a clique decompostion dataframe and the list of cliques
def get_clique_decomposition(mol_smiles, outputs= None, output_name = 'output'):
# Generating Cliques
vocab=cf.Vocabulary(mol_smiles)
size=len(vocab)
vocabl = list(vocab)
MolDict=cf.Vocab2Cat(vocab)
clustersTR=cf.Clusters(mol_smiles)
catTR=cf.Cluster2Cat(clustersTR,MolDict)
clique_decomposition=cf.Vectorize(catTR,size)
descriptors_df = pd.DataFrame(data=clique_decomposition, columns = [x for x in range(len(vocabl))])
if (outputs != None):
descriptors_df[output_name] = output
return descriptors_df, vocabl
def get_train_test_splits(descriptor_df, output,KFold):
# Generating train test splits
splits = {'xtrain':[],
'xtest':[],
'ytrain':[],
'ytest':[]}
for train_index, test_index in KFold.split(descriptor_df.iloc[:,:],output):
splits['xtrain'].append(descriptor_df.iloc[train_index,:])
splits['xtest'].append(descriptor_df.iloc[test_index,:])
splits['ytrain'].append(output.iloc[train_index])
splits['ytest'].append(output.iloc[test_index])
return splits
def get_average_history(history_list):
average_history = np.zeros(len(history_list[0]))
for kfold in history_list: # loop through KFolds
for epoch_index, epoch_hist in zip(range(len(kfold)), kfold): # loop through epochs
average_history[epoch_index] += epoch_hist
average_history = average_history/5
return average_history
def get_std_history(history_list):
std_history = np.zeros(len(history_list[0]))
for x in range(len(history_list[0])):
epoch_i_values = [] # list to store loss at epoch i across k folds
for fold in history_list: # Loop through kfold
epoch_i_values.append(fold[x])
std_history[x] = np.std(epoch_i_values)
return std_history
def work_up(GridSearchCVKerasResults):
train_mae_averages = []
train_mae_stds = []
train_mae_min = []
train_mse_averages = []
train_mse_stds = []
train_mse_min = []
test_mae_averages = []
test_mae_stds = []
test_mae_min = []
test_mse_averages = []
test_mse_min = []
test_mse_stds =[]
for row in GridSearchCVKerasResults.index:
train_mae_list = GridSearchCVKerasResults.loc[row,['train_mae_hist_1','train_mae_hist_2','train_mae_hist_3','train_mae_hist_4','train_mae_hist_5']]
train_mse_list = GridSearchCVKerasResults.loc[row,['train_mse_hist_1','train_mse_hist_2','train_mse_hist_3','train_mse_hist_4','train_mse_hist_5']]
test_mae_list = GridSearchCVKerasResults.loc[row,['test_mae_hist_1','test_mae_hist_2','test_mae_hist_3','test_mae_hist_4','test_mae_hist_5']]
test_mse_list = GridSearchCVKerasResults.loc[row,['test_mse_hist_1','test_mse_hist_2','test_mse_hist_3','test_mse_hist_4','test_mse_hist_5']]
train_mae_averages.append(get_average_history(train_mae_list))
train_mse_averages.append(get_average_history(train_mse_list))
test_mae_averages.append(get_average_history(test_mae_list))
test_mse_averages.append(get_average_history(test_mse_list))
train_mae_stds.append(get_std_history(train_mae_list))
train_mse_stds.append(get_std_history(train_mse_list))
test_mae_stds.append(get_std_history(test_mae_list))
test_mse_stds.append(get_std_history(test_mse_list))
for train_mae, train_mse, test_mae, test_mse in zip(train_mae_averages, train_mse_averages, test_mae_averages, test_mse_averages):
train_mae_min.append(min(train_mae))
train_mse_min.append(min(train_mse))
test_mae_min.append(min(test_mae))
test_mse_min.append(min(test_mse))
GridSearchCVKerasResults['train_mae_average'] = train_mae_averages
GridSearchCVKerasResults['train_mse_average'] = train_mse_averages
GridSearchCVKerasResults['test_mae_average'] = test_mae_averages
GridSearchCVKerasResults['test_mse_average'] = test_mse_averages
GridSearchCVKerasResults['train_mae_std'] = train_mae_stds
GridSearchCVKerasResults['train_mse_std'] = train_mse_stds
GridSearchCVKerasResults['test_mae_std'] = test_mae_stds
GridSearchCVKerasResults['test_mse_std'] = test_mse_stds
GridSearchCVKerasResults['train_mae_min'] = train_mae_min
GridSearchCVKerasResults['train_mse_min'] = train_mse_min
GridSearchCVKerasResults['test_mae_min'] = test_mae_min
GridSearchCVKerasResults['test_mse_min'] = test_mse_min
return GridSearchCVKerasResults
def get_best_model_index_mse(worked_up_results):
best_ind = worked_up_results.loc[worked_up_results['test_mse_min'] == min(worked_up_results['test_mse_min'])].index[0]
return best_ind
def refit_best_model(worked_up_results, splits, scaler,unscaled_outputs):
scaler.fit(np.array(unscaled_outputs).reshape(-1,1))
best_ind = get_best_model_index_mse(worked_up_results)
best_arch = worked_up_results['architecture'][best_ind]
best_acti = worked_up_results['activation'][best_ind]
best_opti = worked_up_results['optimiser'][best_ind]
df = pd.DataFrame(worked_up_results['test_mse_average'][best_ind])
best_epoch = df.loc[df[0] == min(df[0])].index[0]
tr_pred_list = []
te_pred_list = []
for xtr, xte, ytr, yte in zip(splits['xtrain'],splits['xtest'],
splits['ytrain'],splits['ytest']):
best_model = build_model(xtr, architecture=best_arch, activation=best_acti, optimiser=best_opti)
best_model.fit(xtr,ytr,validation_data = [xte,yte],epochs = best_epoch,verbose = 0)
tr_pred_list.append(best_model.predict(xtr))
te_pred_list.append(best_model.predict(xte))
tr_pred_inv_tr_list = []
te_pred_inv_tr_list = []
ytr_inv_tr_list = []
yte_inv_tr_list = []
for tr_pred, te_pred, ytr,yte in zip(tr_pred_list,te_pred_list,splits['ytrain'],splits['ytest']):
tr_pred_inv_tr_list.append(scaler.inverse_transform(tr_pred))
te_pred_inv_tr_list.append(scaler.inverse_transform(te_pred))
ytr_inv_tr_list.append(scaler.inverse_transform(ytr))
yte_inv_tr_list.append(scaler.inverse_transform(yte))
return tr_pred_inv_tr_list, te_pred_inv_tr_list, ytr_inv_tr_list, yte_inv_tr_list
def get_GPModel(data_splits,scaler,LS,optimize=0,method='lbfgsb',restarts=0,LS_bounds=[1e-5,1e5],noise=1e-5):
N=len(data_splits['xtrain'])
LS_opt=[]
predTR=[]
predTE=[]
for i in range(0,N):
xtrain=np.array(data_splits['xtrain'][i])
xtest=np.array(data_splits['xtest'][i])
ytrain=np.array(data_splits['ytrain'][i]).reshape(-1,1)
ytest=np.array(data_splits['ytest'][i]).reshape(-1,1)
# Dimension of our descriptor
D=xtrain.shape[1]
kernel = GPy.kern.RBF(input_dim=D,ARD=True,variance=1,lengthscale=LS[i])
m=GPy.models.GPRegression(xtrain,ytrain,kernel)
m.constrain_bounded(LS_bounds[0],LS_bounds[1]) # bound lengthscales
m.kern.variance.fix() # fixing the variance
if noise>0:
m.likelihood.variance.fix(noise) # fixing the noise param
if optimize==1:
m.optimize(optimizer=method,messages=False,max_iters=10000)
if restarts>0:
m.optimize_restarts(optimizer=method,messages=False,max_iters=10000,num_restarts=restarts,verbose=False)
LS_opt.append(np.array(m.rbf.lengthscale)) # Append optimized len
#print(m)
# Appending model predictions
predTR.append(scaler.inverse_transform(m.predict(xtrain)))
predTE.append(scaler.inverse_transform(m.predict(xtest)))
return predTR,predTE,LS_opt
def GP_RFplot(data_splits,predTR,predTE,scaler,data_name='Hepatocytes',model='RF',alpha=0.35):
N=len(data_splits['ytrain'])
TR_PRED=[]
TR_EXP=[]
TE_PRED=[]
TE_EXP=[]
fig = plt.figure(figsize=[3,3],edgecolor='k',dpi = 200)
#sns.set_style("white")
for i in range(0,N):
TR_EXP=TR_EXP+list(scaler.inverse_transform(data_splits['ytrain'][i]))
TE_EXP=TE_EXP+list(scaler.inverse_transform(data_splits['ytest'][i]))
if model=='GP':
TR_PRED=TR_PRED+list(predTR[i][0])
TE_PRED=TE_PRED+list(predTE[i][0])
else:
TR_PRED=TR_PRED+list(predTR[i])
TE_PRED=TE_PRED+list(predTE[i])
plt.scatter(TR_EXP,TR_PRED,label='Train Set',s=15)
plt.scatter(TE_EXP,TE_PRED,label='Test Set',s=15,alpha=alpha)
plt.legend(fontsize=8, prop={'size':6})
plt.xlabel('Experimental',fontsize=8)
plt.ylabel('Model Prediction',fontsize=8)
if model=='GP':
acc=np.linspace(min(predTR[0][0])-0.15,max(predTR[0][0]+0.15),1000)
plt.plot(acc,acc,label = 'Accuracy',color='green',linewidth=1)
plt.title('%s Gaussian Process' %data_name,fontsize = 8)
else:
acc=np.linspace(min(predTR[0])-0.15,max(predTR[0])+0.15,1000)
plt.plot(acc,acc,label = 'Accuracy',color='green',linewidth=1)
plt.title('%s Random Forests' %data_name,fontsize = 8)
return None
def GP_MSE(data_splits,predTR,predTE,scaler):
TR_mse=[]
TE_mse=[]
for i in range(0,5):
TR_mse.append(mse(scaler.inverse_transform(data_splits['ytrain'][i]),predTR[i][0,:]))
TE_mse.append(mse(scaler.inverse_transform(data_splits['ytest'][i]),predTE[i][0,:]))
mse_df=pd.DataFrame(data=[TR_mse,TE_mse]).T
mse_df.columns=['train_mse','test_mse']
print(mse_df)
return None
# Borrowed this code from www.geeksforgeeks.org
# Sorts a list based on a second list
def sort_list(list1,list2):
zipped_pairs=zip(list2, list1)
z = [x for _, x in sorted(zipped_pairs)]
return z
def plot_all_lengthscales(lengthscales,xlim,ylim,title):
N=len(lengthscales[0])
fig = plt.figure(figsize=[25,15],edgecolor='k',dpi = 200)
sns.set_style("white")
xindex=np.arange(N)
width=2.5
plt.bar(xindex,lengthscales[0],width,color='navy',label='Split 1')
plt.bar(xindex,lengthscales[1],width,color='red',alpha=0.9,label='Split 2')
plt.bar(xindex,lengthscales[2],width,color='green',alpha=1.0,label='Split 3')
plt.bar(xindex,lengthscales[3],width,color='dodgerblue',alpha=0.8,label='Split 4')
plt.bar(xindex,lengthscales[4],width,color='gold',alpha=0.7,label='Split 5')
plt.ylim(ylim)
plt.xlim(xlim)
plt.xlabel('Lengthscale Index',fontsize=20)
plt.ylabel('Lengthscale Value',fontsize=20)
plt.legend(loc='best',prop={'size':20})
plt.title('%s Lengthscale Comparison by Split' %title,fontsize=25)
return None
def plot_particular_lengthscales(lengthscales,indices,title):
N=len(lengthscales[0])
fig=plt.figure(figsize=[20,8],edgecolor='k',dpi = 200)
ax=plt.axes()
sns.set_style("white")
width=2.5
ax.bar(indices,lengthscales[0][indices],width,color='navy',label='Split 1')
ax.bar(indices,lengthscales[1][indices],width,color='red',alpha=0.9,label='Split 2')
ax.bar(indices,lengthscales[2][indices],width,color='green',alpha=1.0,label='Split 3')
ax.bar(indices,lengthscales[3][indices],width,color='dodgerblue',alpha=0.8,label='Split 4')
ax.bar(indices,lengthscales[4][indices],width,color='yellow',alpha=0.6,label='Split 5')
if max(max(lengthscales[0][indices]),max(lengthscales[1][indices]),max(lengthscales[2][indices]),max(lengthscales[3][indices]),max(lengthscales[4][indices]))<800:
plt.ylim(0,max(max(lengthscales[0][indices]),max(lengthscales[1][indices]),max(lengthscales[2][indices]),max(lengthscales[3][indices]),max(lengthscales[4][indices]))*1.05)
else:
plt.ylim(0,max(max(lengthscales[0][indices]),max(lengthscales[1][indices]),max(lengthscales[2][indices]),max(lengthscales[3][indices]),max(lengthscales[4][indices]))*0.25)
plt.xlim(-1,max(np.array(indices))+5)
plt.xlabel('Lengthscale Index',fontsize=20)
plt.ylabel('Lengthscale Value',fontsize=20)
plt.legend(loc='best',prop={'size':20})
plt.title('%s Lengthscale Comparison by Split' %title,fontsize=25)
ax.set_xticks(indices)
return None
def mean_lengthscale(lengthscales):
mean_LS=np.mean(lengthscales,0)
std_LS=np.std(lengthscales,0)
return mean_LS,std_LS
def sort_lengthscales(lengthscales,k_splits):
N=len(lengthscales[0])
sortLS=[]
for i in range(0,k_splits):
split=lengthscales[i]
indices=split.argsort() # smallest lengthscales (indices in ascending order based on LS value)
sortLS.append(indices) #Lists the lengthscale ranks
meanrank=[]
for j in range(0,N):
indexsum=0
for k in range(0,k_splits):
indexsum+=np.where(sortLS[k]==j)[0][0]
mean=indexsum/5
meanrank.append(mean)
meanrank=np.array(meanrank)
sortedcliques=meanrank.argsort() #Sorts the indices of ls from smallest to largest
return sortedcliques,meanrank
def get_LS_df(indices,vocab,lengthscales):
class dictionary(dict):
# __init__ function
def __init__(self):
self=dict()
# Function to add key:value
def add(self, key, value):
self[key]=value
smiles=[vocab[i] for i in indices]
LS_mean=np.take(np.mean(lengthscales,0),indices)
LS_std=np.take(np.std(lengthscales,0),indices)
LSdict=dictionary()
LSdict.add('vocab_index',indices)
LSdict.add('smiles',smiles)
LSdict.add('mean_LS',LS_mean)
LSdict.add('std_LS',LS_std)
for j in np.arange(0,len(lengthscales)):
LSdict.add('split%s'%(j+1),np.take(lengthscales[j],indices))
return pd.DataFrame(LSdict)
def get_RFmodel(data_splits,scaler):
# Instantiate model with 10000 decision trees
N=len(data_splits['xtrain'])
rf=RandomForestRegressor(n_estimators=10000,random_state=10)
RFpredTR=[]
RFpredTE=[]
RFgini=[]
for i in range(0,N):
xtrain=np.array(data_splits['xtrain'][i])
xtest=np.array(data_splits['xtest'][i])
ytrain=np.array(data_splits['ytrain'][i]).reshape(-1,1)
ytest=np.array(data_splits['ytest'][i]).reshape(-1,1)
#Train the model on training data
rf.fit(xtrain,ytrain)
#Use the forest's predict method on the test data
RFpredTR.append(scaler.inverse_transform(rf.predict(xtrain)))
RFpredTE.append(scaler.inverse_transform(rf.predict(xtest)))
RFgini.append(rf.feature_importances_)
return RFpredTR,RFpredTE,RFgini
def RF_MSE(data_splits,predTR,predTE,scaler):
N=len(data_splits['ytrain'])
# MSE analysis
TR_mse_RF=[]
TE_mse_RF=[]
for i in range(0,N):
TR_mse_RF.append(mse(scaler.inverse_transform(data_splits['ytrain'][i]),predTR[i]))
TE_mse_RF.append(mse(scaler.inverse_transform(data_splits['ytest'][i]),predTE[i]))
mse_RF_df=pd.DataFrame(data=[TR_mse_RF,TE_mse_RF]).T
mse_RF_df.columns=['train_mse','test_mse']
print(mse_RF_df)
return None
def sort_gini(gini,k_splits):
N=len(gini[0])
sorted_gini=[]
for i in range(0,k_splits):
split=gini[i]
indices=split.argsort() #Sorts indices of gini values from smallest to largest
sorted_gini.append(indices)
indexmean_gini=[]
for j in range(0,N):
indexsum=0
for k in range(0,k_splits):
indexsum+=np.where(sorted_gini[k]==j)[0][0]
mean=indexsum/5
indexmean_gini.append(mean)
indexmean_gini=np.array(indexmean_gini) # Actually use this list to sort the cliques
#Sorted array of cliques from smallest gini to largest
gini_rank=indexmean_gini.argsort()[::-1]
return sorted_gini,gini_rank
def get_gini_df(indices,vocab,gini):
class dictionary(dict):
# __init__ function
def __init__(self):
self=dict()
# Function to add key:value
def add(self, key, value):
self[key]=value
smiles=[vocab[i] for i in indices]
gini_mean=np.take(np.mean(gini,0),indices)
gini_std=np.take(np.std(gini,0),indices)
GINIdict=dictionary()
GINIdict.add('vocab_index',indices)
GINIdict.add('smiles',smiles)
GINIdict.add('mean_gini',gini_mean)
GINIdict.add('std_gini',gini_std)
for j in np.arange(0,len(gini)):
GINIdict.add('split%s'%(j+1),np.take(gini[j],indices))
return pd.DataFrame(GINIdict)
def plot_gini(gini_df,dataname='Lipo'):
xlabels=list(gini_df['vocab_index'])
gini_std=list(gini_df['std_gini'])
gini_mean=list(gini_df['mean_gini'])
x_position=np.arange(0,len(xlabels))
#fig = plt.figure(figsize=[3,3],edgecolor='k',dpi = 200)
fig,ax=plt.subplots(figsize=[10,8],edgecolor='k',dpi = 200)
ax.bar(x_position,gini_mean,yerr=gini_std,align='center',alpha=1.0)#,ecolor='black')
ax.set_ylabel('Mean gini value')
ax.set_xlabel('Clique Index')
ax.set_xticks(x_position)
ax.set_xticklabels(xlabels)
ax.set_title('Mean/Std of %s RF gini values' %dataname)
ax.yaxis.grid(True)
return None
# Lets make some predictions on the various dataset/descriptor combinations using the best models as determined by the
# optimisation process
def make_predictions(unscaled_output, splits, best_model_results, scaler):
results = {}
results['ytr_scaled'] = [] # place to store scaled train set outputs for splits 1-5
results['yte_scaled'] = [] # place to store scaled test set outputs for splits 1-5
results['tr_preds_scaled'] = [] # place to store scaled train predictions for splits 1-5
results['te_preds_scaled'] = [] # place to store scaled test predictions for splits 1-5
results['ytr'] = [] # place to store train set outputs for splits 1-5
results['yte'] = [] # place to store test set outputs for splits 1-5
results['tr_preds'] = [] # place to store normal output space train predictions for splits 1-5
results['te_preds'] = [] # place to store normal output space test predictions for splits 1-5
best_arch = best_model_results['architecture'].values[0] # Getting best architecture
best_acti = best_model_results['activation'].values[0] # Getting best activation function
best_opti = best_model_results['optimiser'].values[0] # Getting best optimiser
ind = np.argmin(best_model_results['test_mse_average'].values[0])
best_epoch = best_model_results['epoch'].values[0][ind] # Getting best number of epochs
scaler.fit(np.array(unscaled_output).reshape(-1,1)) # To transform scaled splits back to original output space
for xtr, xte, ytr,yte, index in zip(splits['xtrain'],splits['xtest'],splits['ytrain'],splits['ytest'],
[x for x in range(1,6,1)]):
model = build_model(xtr, best_arch, best_acti, best_opti)
model.fit(xtr,ytr, validation_data = [xte,yte], epochs = best_epoch, verbose = 0)
tr_pred = model.predict(xtr)
te_pred = model.predict(xte)
results['ytr_scaled'].append(ytr)
results['yte_scaled'].append(yte)
results['tr_preds_scaled'].append(tr_pred)
results['te_preds_scaled'].append(te_pred)
results['ytr'].append(scaler.inverse_transform(np.array(ytr).reshape(-1,1)))
results['yte'].append(scaler.inverse_transform(np.array(yte).reshape(-1,1)))
results['tr_preds'].append(scaler.inverse_transform(tr_pred))
results['te_preds'].append(scaler.inverse_transform(te_pred))
return pd.DataFrame(results)
def plot_results(predictions,fig_title):
fig, (ax1,ax2) = plt.subplots(1,2,figsize=[12,6],dpi=200)
plt.suptitle(fig_title)
ax1.set_ylabel('Predicted Value')
ax1.set_xlabel('Experimental Value')
# Plotting predicted vs experimental
acc = np.linspace(min(predictions['ytr'][0]),max(predictions['ytr'][0]),1000)
ax1.plot(acc,acc)
for ytr,tr_pred in zip(predictions['ytr'],predictions['tr_preds']):
tr_scat = ax1.scatter(ytr,tr_pred,label='Train Set',color='Blue',alpha=1)
for yte,te_pred in zip(predictions['yte'],predictions['te_preds']):
te_scat = ax1.scatter(yte,te_pred,label='Test Set',color='orange',alpha=0.5)
ax1.legend([tr_scat,te_scat],['Train Predictions','Test Predictions'])
# Plotting distribution of errors
train_errors = []
test_errors = []
for ytr_split, tr_pred_split in zip(predictions['ytr'],predictions['tr_preds']):
for ytr,tr_pred in zip(ytr_split,tr_pred_split):
train_errors.append(float((ytr-tr_pred)))
for yte_split, te_pred_split in zip(predictions['yte'],predictions['te_preds']):
for yte,te_pred in zip(yte_split,te_pred_split):
test_errors.append(float((yte-te_pred)))
sns.distplot(train_errors, label="Train Errors")
sns.distplot(test_errors, label="Test Errors")
ax2.legend()
ax2.set_xlabel('Error')
ax2.set_ylabel('Counts')
# Function that returns average mse, mae, and Pearson's R for a set of predictions, in form of dataframe
def get_ave_metrics(predictions,name):
mse_train_list = []
mae_train_list = []
r_train_list = []
mse_test_list = []
mae_test_list = []
r_test_list = []
for ytr,tr_pred,yte,te_pred in zip(predictions['ytr'],predictions['tr_preds'],
predictions['yte'],predictions['te_preds']):
mse_train_list.append(mse(ytr,tr_pred))
mae_train_list.append(mae(ytr,tr_pred))
r_train_list.append(pearsonr(ytr,tr_pred)[0])
mse_test_list.append(mse(yte,te_pred))
mae_test_list.append(mae(yte,te_pred))
r_test_list.append(pearsonr(yte,te_pred)[0])
results = {'mse_train_ave':0,
'mse_train_std':0,
'mae_train_ave':0,
'mae_train_std':0,
'pearsonr_train_ave':0,
'pearsonr_train_std':0,
'mse_test_ave':0,
'mse_test_std':0,
'mae_test_ave':0,
'mae_test_std':0,
'pearsonr_test_ave':0,
'pearsonr_test_std':0}
results['mse_train_ave']=np.average(mse_train_list)
results['mse_train_std']=np.std(mse_train_list)
results['mae_train_ave']=np.average(mae_train_list)
results['mae_train_std']=np.std(mae_train_list)
r_train_list = np.array(r_train_list).reshape(-1)
results['pearsonr_train_ave']=np.average(r_train_list)
results['pearsonr_train_std']=np.std(r_train_list)
results['mse_test_ave']=np.average(mse_test_list)
results['mse_test_std']=np.std(mse_test_list)
results['mae_test_ave']=np.average(mae_test_list)
results['mae_test_std']=np.std(mae_test_list)
r_test_list = np.array(r_test_list).reshape(-1)
results['pearsonr_test_ave']=np.average(r_test_list)
results['pearsonr_test_std']=np.std(r_test_list)
return pd.DataFrame(results,index=[name]).T
def plot_bar(metrics_df,metric):
fig = plt.figure(figsize=[16,12])
plt.bar(metrics_df.columns,metrics_df.loc[metric+'_ave',:],edgecolor='k',color='orange',
yerr=metrics_df.loc[metric+'_std',:])
plt.ylabel(metric,fontsize=20)
plt.yticks(fontsize=20)
plt.xticks(fontsize=12 )
plt.title(metric+' across each dataset/descriptor combination',fontsize=20)
<file_sep>import numpy as np
import pandas as pd
from numpy.linalg import eigvalsh,eigh
from rdkit import Chem
from rdkit.Chem.rdmolops import GetAdjacencyMatrix
from rdkit.Chem.EState import Fingerprinter
from rdkit.Chem import Descriptors
from rdkit.Chem.rdmolops import RDKFingerprint
from rdkit.Chem import AllChem
from rdkit.Chem.Crippen import MolLogP,MolMR
from sklearn.gaussian_process import GaussianProcessRegressor
from sklearn.gaussian_process.kernels import RBF, ConstantKernel as C
from sklearn.gaussian_process.kernels import Matern, WhiteKernel, ConstantKernel
import scipy as sp
from sklearn.base import BaseEstimator
import matplotlib.pyplot as plt
import seaborn as sns
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import scale,normalize
from sklearn.model_selection import RepeatedKFold
import collections
# Flatten these nasty nested lists...
def flatten(x):
if isinstance(x, collections.Iterable):
return [a for i in x for a in flatten(i)]
else:
return [x]
def t3DMOL(mole):
return AllChem.EmbedMolecule(mole,useRandomCoords=True)
def t3DMOLES(moles):
vectt3DMOL=np.vectorize(t3DMOL)
return vectt3DMOL(moles)
def UFF(mole):
return AllChem.UFFOptimizeMolecule(mole,maxIters=1000)
def UFFSS(moles):
vecUFF=np.vectorize(UFF)
return vecUFF(moles)
# Function to get some standard descriptors via RDKit
def STDD(molecules):
std_descriptors=[]
i=0
for m in molecules:
print("Dealing with molecule n. ",i," of ",len(molecules))
i=i+1
desc=[]
# 2D
desc.append(Chem.GraphDescriptors.BalabanJ(m)) # Chem. Phys. Lett. 89:399-404 (1982)
desc.append(Chem.GraphDescriptors.BertzCT(m)) # J. Am. Chem. Soc. 103:3599-601 (1981)
desc.append(Chem.GraphDescriptors.HallKierAlpha(m)) # Rev. Comput. Chem. 2:367-422 (1991)
desc.append(Chem.Crippen.MolLogP(m)) # Wildman and Crippen JCICS 39:868-73 (1999)
desc.append(Chem.Crippen.MolMR(m)) # Wildman and Crippen JCICS 39:868-73 (1999)
desc.append(Chem.Descriptors.ExactMolWt(m))
desc.append(Chem.Descriptors.FpDensityMorgan1(m))
desc.append(Chem.Descriptors.MaxPartialCharge(m))
desc.append(Chem.Descriptors.NumRadicalElectrons(m))
desc.append(Chem.Descriptors.NumValenceElectrons(m))
desc.append(Chem.Lipinski.FractionCSP3(m))
desc.append(Chem.Lipinski.HeavyAtomCount(m))
desc.append(Chem.Lipinski.NumHAcceptors(m))
desc.append(Chem.Lipinski.NumAromaticRings(m))
desc.append(Chem.Lipinski.NHOHCount(m))
desc.append(Chem.Lipinski.NOCount(m))
desc.append(Chem.Lipinski.NumAliphaticCarbocycles(m))
desc.append(Chem.Lipinski.NumAliphaticHeterocycles(m))
desc.append(Chem.Lipinski.NumAliphaticRings(m))
desc.append(Chem.Lipinski.NumAromaticCarbocycles(m))
desc.append(Chem.Lipinski.NumAromaticHeterocycles(m))
desc.append(Chem.Lipinski.NumHDonors(m))
desc.append(Chem.Lipinski.NumHeteroatoms(m))
desc.append(Chem.Lipinski.NumRotatableBonds(m))
desc.append(Chem.Lipinski.NumSaturatedCarbocycles(m))
desc.append(Chem.Lipinski.NumSaturatedHeterocycles(m))
desc.append(Chem.Lipinski.NumSaturatedRings(m))
desc.append(Chem.Lipinski.RingCount(m))
desc.append(Chem.rdMolDescriptors.CalcTPSA(m))
desc.append(Chem.rdMolDescriptors.CalcTPSA(m)) # J. Med. Chem. 43:3714-7, (2000)
desc.append(Chem.MolSurf.LabuteASA(m)) # J. Mol. Graph. Mod. 18:464-77 (2000)
# 3D
desc.append(Chem.rdMolDescriptors.CalcAUTOCORR2D(m))
desc.append(Chem.rdMolDescriptors.CalcAUTOCORR3D(m))
desc.append(Chem.rdMolDescriptors.CalcAsphericity(m))
desc.append(Chem.rdMolDescriptors.CalcChi0n(m))
desc.append(Chem.rdMolDescriptors.CalcChi0v(m))
desc.append(Chem.rdMolDescriptors.CalcChi1n(m))
desc.append(Chem.rdMolDescriptors.CalcChi1v(m))
desc.append(Chem.rdMolDescriptors.CalcChi2n(m))
desc.append(Chem.rdMolDescriptors.CalcChi2v(m))
desc.append(Chem.rdMolDescriptors.CalcChi3v(m))
desc.append(Chem.rdMolDescriptors.CalcChi4n(m))
desc.append(Chem.rdMolDescriptors.CalcChi4v(m))
desc.append(Chem.rdMolDescriptors.CalcCrippenDescriptors(m))
desc.append(Chem.rdMolDescriptors.CalcEccentricity(m))
desc.append(Chem.rdMolDescriptors.CalcExactMolWt(m))
desc.append(Chem.rdMolDescriptors.CalcFractionCSP3(m))
#desc.append(Chem.rdMolDescriptors.CalcGETAWAY(m))
desc.append(Chem.rdMolDescriptors.CalcHallKierAlpha(m))
desc.append(Chem.rdMolDescriptors.CalcInertialShapeFactor(m))
desc.append(Chem.rdMolDescriptors.CalcKappa1(m))
desc.append(Chem.rdMolDescriptors.CalcKappa2(m))
desc.append(Chem.rdMolDescriptors.CalcKappa3(m))
desc.append(Chem.rdMolDescriptors.CalcLabuteASA(m))
desc.append(Chem.rdMolDescriptors.CalcMORSE(m))
desc.append(Chem.rdMolDescriptors.CalcNPR1(m))
desc.append(Chem.rdMolDescriptors.CalcNPR2(m))
desc.append(Chem.rdMolDescriptors.CalcNumAliphaticCarbocycles(m))
desc.append(Chem.rdMolDescriptors.CalcNumAliphaticHeterocycles(m))
desc.append(Chem.rdMolDescriptors.CalcNumAliphaticRings(m))
desc.append(Chem.rdMolDescriptors.CalcNumAmideBonds(m))
desc.append(Chem.rdMolDescriptors.CalcNumAromaticCarbocycles(m))
desc.append(Chem.rdMolDescriptors.CalcNumAromaticHeterocycles(m))
desc.append(Chem.rdMolDescriptors.CalcNumAromaticRings(m))
#desc.append(Chem.rdMolDescriptors.CalcNumAtomStereoCenters(m))
desc.append(Chem.rdMolDescriptors.CalcNumBridgeheadAtoms(m))
desc.append(Chem.rdMolDescriptors.CalcNumHBA(m))
desc.append(Chem.rdMolDescriptors.CalcNumHBD(m))
desc.append(Chem.rdMolDescriptors.CalcNumHeteroatoms(m))
desc.append(Chem.rdMolDescriptors.CalcNumHeterocycles(m))
desc.append(Chem.rdMolDescriptors.CalcNumLipinskiHBA(m))
desc.append(Chem.rdMolDescriptors.CalcNumLipinskiHBD(m))
desc.append(Chem.rdMolDescriptors.CalcNumRings(m))
desc.append(Chem.rdMolDescriptors.CalcNumRotatableBonds(m))
desc.append(Chem.rdMolDescriptors.CalcNumSaturatedCarbocycles(m))
desc.append(Chem.rdMolDescriptors.CalcNumSaturatedHeterocycles(m))
desc.append(Chem.rdMolDescriptors.CalcNumSaturatedRings(m))
desc.append(Chem.rdMolDescriptors.CalcNumSpiroAtoms(m))
desc.append(Chem.rdMolDescriptors.CalcPBF(m))
desc.append(Chem.rdMolDescriptors.CalcPMI1(m))
desc.append(Chem.rdMolDescriptors.CalcPMI2(m))
desc.append(Chem.rdMolDescriptors.CalcPMI3(m))
desc.append(Chem.rdMolDescriptors.CalcRDF(m))
desc.append(Chem.rdMolDescriptors.CalcRadiusOfGyration(m))
desc.append(Chem.rdMolDescriptors.CalcSpherocityIndex(m))
desc.append(Chem.rdMolDescriptors.CalcTPSA(m))
desc.append(Chem.rdMolDescriptors.CalcWHIM(m))
desc=flatten(desc)
std_descriptors.append(desc)
return std_descriptors
def STD_ONE(m):
std_descriptors=[]
i=0
desc=[]
# 2D
desc.append(Chem.GraphDescriptors.BalabanJ(m)) # Chem. Phys. Lett. 89:399-404 (1982)
desc.append(Chem.GraphDescriptors.BertzCT(m)) # J. Am. Chem. Soc. 103:3599-601 (1981)
desc.append(Chem.GraphDescriptors.HallKierAlpha(m)) # Rev. Comput. Chem. 2:367-422 (1991)
desc.append(Chem.Crippen.MolLogP(m)) # Wildman and Crippen JCICS 39:868-73 (1999)
desc.append(Chem.Crippen.MolMR(m)) # Wildman and Crippen JCICS 39:868-73 (1999)
desc.append(Chem.Descriptors.ExactMolWt(m))
desc.append(Chem.Descriptors.FpDensityMorgan1(m))
desc.append(Chem.Descriptors.MaxPartialCharge(m))
desc.append(Chem.Descriptors.NumRadicalElectrons(m))
desc.append(Chem.Descriptors.NumValenceElectrons(m))
desc.append(Chem.Lipinski.FractionCSP3(m))
desc.append(Chem.Lipinski.HeavyAtomCount(m))
desc.append(Chem.Lipinski.NumHAcceptors(m))
desc.append(Chem.Lipinski.NumAromaticRings(m))
desc.append(Chem.Lipinski.NHOHCount(m))
desc.append(Chem.Lipinski.NOCount(m))
desc.append(Chem.Lipinski.NumAliphaticCarbocycles(m))
desc.append(Chem.Lipinski.NumAliphaticHeterocycles(m))
desc.append(Chem.Lipinski.NumAliphaticRings(m))
desc.append(Chem.Lipinski.NumAromaticCarbocycles(m))
desc.append(Chem.Lipinski.NumAromaticHeterocycles(m))
desc.append(Chem.Lipinski.NumHDonors(m))
desc.append(Chem.Lipinski.NumHeteroatoms(m))
desc.append(Chem.Lipinski.NumRotatableBonds(m))
desc.append(Chem.Lipinski.NumSaturatedCarbocycles(m))
desc.append(Chem.Lipinski.NumSaturatedHeterocycles(m))
desc.append(Chem.Lipinski.NumSaturatedRings(m))
desc.append(Chem.Lipinski.RingCount(m))
desc.append(Chem.rdMolDescriptors.CalcTPSA(m))
desc.append(Chem.rdMolDescriptors.CalcTPSA(m)) # J. Med. Chem. 43:3714-7, (2000)
desc.append(Chem.MolSurf.LabuteASA(m)) # J. Mol. Graph. Mod. 18:464-77 (2000)
# 3D
desc.append(Chem.rdMolDescriptors.CalcAUTOCORR2D(m))
desc.append(Chem.rdMolDescriptors.CalcAUTOCORR3D(m))
desc.append(Chem.rdMolDescriptors.CalcAsphericity(m))
desc.append(Chem.rdMolDescriptors.CalcChi0n(m))
desc.append(Chem.rdMolDescriptors.CalcChi0v(m))
desc.append(Chem.rdMolDescriptors.CalcChi1n(m))
desc.append(Chem.rdMolDescriptors.CalcChi1v(m))
desc.append(Chem.rdMolDescriptors.CalcChi2n(m))
desc.append(Chem.rdMolDescriptors.CalcChi2v(m))
desc.append(Chem.rdMolDescriptors.CalcChi3v(m))
desc.append(Chem.rdMolDescriptors.CalcChi4n(m))
desc.append(Chem.rdMolDescriptors.CalcChi4v(m))
desc.append(Chem.rdMolDescriptors.CalcCrippenDescriptors(m))
desc.append(Chem.rdMolDescriptors.CalcEccentricity(m))
desc.append(Chem.rdMolDescriptors.CalcExactMolWt(m))
desc.append(Chem.rdMolDescriptors.CalcFractionCSP3(m))
#desc.append(Chem.rdMolDescriptors.CalcGETAWAY(m))
desc.append(Chem.rdMolDescriptors.CalcHallKierAlpha(m))
desc.append(Chem.rdMolDescriptors.CalcInertialShapeFactor(m))
desc.append(Chem.rdMolDescriptors.CalcKappa1(m))
desc.append(Chem.rdMolDescriptors.CalcKappa2(m))
desc.append(Chem.rdMolDescriptors.CalcKappa3(m))
desc.append(Chem.rdMolDescriptors.CalcLabuteASA(m))
desc.append(Chem.rdMolDescriptors.CalcMORSE(m))
desc.append(Chem.rdMolDescriptors.CalcNPR1(m))
desc.append(Chem.rdMolDescriptors.CalcNPR2(m))
desc.append(Chem.rdMolDescriptors.CalcNumAliphaticCarbocycles(m))
desc.append(Chem.rdMolDescriptors.CalcNumAliphaticHeterocycles(m))
desc.append(Chem.rdMolDescriptors.CalcNumAliphaticRings(m))
desc.append(Chem.rdMolDescriptors.CalcNumAmideBonds(m))
desc.append(Chem.rdMolDescriptors.CalcNumAromaticCarbocycles(m))
desc.append(Chem.rdMolDescriptors.CalcNumAromaticHeterocycles(m))
desc.append(Chem.rdMolDescriptors.CalcNumAromaticRings(m))
#desc.append(Chem.rdMolDescriptors.CalcNumAtomStereoCenters(m))
desc.append(Chem.rdMolDescriptors.CalcNumBridgeheadAtoms(m))
desc.append(Chem.rdMolDescriptors.CalcNumHBA(m))
desc.append(Chem.rdMolDescriptors.CalcNumHBD(m))
desc.append(Chem.rdMolDescriptors.CalcNumHeteroatoms(m))
desc.append(Chem.rdMolDescriptors.CalcNumHeterocycles(m))
desc.append(Chem.rdMolDescriptors.CalcNumLipinskiHBA(m))
desc.append(Chem.rdMolDescriptors.CalcNumLipinskiHBD(m))
desc.append(Chem.rdMolDescriptors.CalcNumRings(m))
desc.append(Chem.rdMolDescriptors.CalcNumRotatableBonds(m))
desc.append(Chem.rdMolDescriptors.CalcNumSaturatedCarbocycles(m))
desc.append(Chem.rdMolDescriptors.CalcNumSaturatedHeterocycles(m))
desc.append(Chem.rdMolDescriptors.CalcNumSaturatedRings(m))
desc.append(Chem.rdMolDescriptors.CalcNumSpiroAtoms(m))
desc.append(Chem.rdMolDescriptors.CalcPBF(m))
desc.append(Chem.rdMolDescriptors.CalcPMI1(m))
desc.append(Chem.rdMolDescriptors.CalcPMI2(m))
desc.append(Chem.rdMolDescriptors.CalcPMI3(m))
desc.append(Chem.rdMolDescriptors.CalcRDF(m))
desc.append(Chem.rdMolDescriptors.CalcRadiusOfGyration(m))
desc.append(Chem.rdMolDescriptors.CalcSpherocityIndex(m))
desc.append(Chem.rdMolDescriptors.CalcTPSA(m))
desc.append(Chem.rdMolDescriptors.CalcWHIM(m))
desc=flatten(desc)
std_descriptors.append(desc)
return std_descriptors
<file_sep># MSDE Functions
import keras
from keras.layers import Dense, Input
import PYTHON.chemfun as cf
#import PYTHON.chemfun_1 as cf1
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
import seaborn as sns
import datetime
# Tensorflow graph being a nuisance and makes optimisation process slow down as the number of models increases,
# trying to make function that resets graphs after each model training
def ResetGraph():
keras.backend.clear_session()
# Building the model #
def build_model(input,architecture, activation, optimiser):
ResetGraph()
input1 = Input(shape = (len(input.columns),))
a = input1
for layer in architecture:
a = Dense(layer,activation = activation)(a)
output1 = Dense(1,activation = 'linear')(a)
model = keras.models.Model(inputs = input1, outputs = output1)
model.compile(optimizer = optimiser, loss='mean_squared_error', metrics=['mean_absolute_error', 'mean_squared_error'])
return model
def GridSearchCVKeras(xtrain_list, xtest_list, ytrain_list, ytest_list, param_grid):
results = {'epochs':[],
'architecture':[],
'activation':[],
'optimiser':[]}
for x in range(1,len(xtrain_list)+1,1):
results['train_mae_hist_%s'%(x)] = []
results['train_mse_hist_%s'%(x)] = []
results['test_mae_hist_%s'%(x)] = []
results['test_mse_hist_%s'%(x)] = []
num_models = 1
for key in param_grid.keys():
num_models*=len(param_grid[key])
counter = 1
for architecture in param_grid['architecture']: # Grid Search
for activation in param_grid['activation']:
for optimiser in param_grid['optimiser']:
for epoch in param_grid['epochs']:
results['architecture'].append(architecture)
results['activation'].append(activation)
results['optimiser'].append(optimiser)
results['epochs'].append([x for x in range(1,epoch+1,1)])
print('Model Number:',counter,' | Current time:', datetime.datetime.now())
index=1
for xtr, xte, ytr, yte in zip(xtrain_list, xtest_list, ytrain_list, ytest_list):
model = build_model(xtr, architecture, activation, optimiser)
history = model.fit(xtr,ytr, validation_data = [xte,yte],epochs = epoch, verbose = 0)
hist = history.history
results['train_mse_hist_%s'%(index)].append(hist['mean_squared_error'])
results['train_mae_hist_%s'%(index)].append(hist['mean_absolute_error'])
results['test_mse_hist_%s'%(index)].append(hist['val_mean_squared_error'])
results['test_mae_hist_%s'%(index)].append(hist['val_mean_absolute_error'])
ResetGraph()
index += 1
counter+=1
return pd.DataFrame(results)
def get_standard_descriptors(mol_smiles, output = None, output_name = 'output'):
mol_rdkit = cf.SMILES2MOLES(mol_smiles) # Converting smiles to RD-Kit format
mol_rdkit_H = cf1.ADDH_MOLES(mol_rdkit) # Adding hydrogens to molecules
descriptors = []
for m in mol_rdkit_H:
desc = []
desc.append(Chem.GraphDescriptors.BalabanJ(m)) # Chem. Phys. Lett. 89:399-404 (1982)
desc.append(Chem.GraphDescriptors.BertzCT(m)) # J. Am. Chem. Soc. 103:3599-601 (1981)
desc.append(Chem.GraphDescriptors.Ipc(m)) # J. Chem. Phys. 67:4517-33 (1977) # Gives very small numbers #
desc.append(Chem.GraphDescriptors.HallKierAlpha(m)) # Rev. Comput. Chem. 2:367-422 (1991)
desc.append(Chem.Crippen.MolLogP(m)) # Wildman and Crippen JCICS 39:868-73 (1999)
desc.append(Chem.Crippen.MolMR(m)) # Wildman and Crippen JCICS 39:868-73 (1999)
desc.append(Chem.Descriptors.ExactMolWt(m))
desc.append(Chem.Descriptors.FpDensityMorgan1(m))
#desc.append(Chem.Descriptors.MaxPartialCharge(m)) # Returns null value for a molecule in lipophilicity dataset #
desc.append(Chem.Descriptors.NumRadicalElectrons(m))
desc.append(Chem.Descriptors.NumValenceElectrons(m))
desc.append(Chem.Lipinski.FractionCSP3(m))
desc.append(Chem.Lipinski.HeavyAtomCount(m))
desc.append(Chem.Lipinski.NumHAcceptors(m))
desc.append(Chem.Lipinski.NumAromaticRings(m))
desc.append(Chem.Lipinski.NHOHCount(m))
desc.append(Chem.Lipinski.NOCount(m))
desc.append(Chem.Lipinski.NumAliphaticCarbocycles(m))
desc.append(Chem.Lipinski.NumAliphaticHeterocycles(m))
desc.append(Chem.Lipinski.NumAliphaticRings(m))
desc.append(Chem.Lipinski.NumAromaticCarbocycles(m))
desc.append(Chem.Lipinski.NumAromaticHeterocycles(m))
desc.append(Chem.Lipinski.NumHDonors(m))
desc.append(Chem.Lipinski.NumHeteroatoms(m))
desc.append(Chem.Lipinski.NumRotatableBonds(m))
desc.append(Chem.Lipinski.NumSaturatedCarbocycles(m))
desc.append(Chem.Lipinski.NumSaturatedHeterocycles(m))
desc.append(Chem.Lipinski.NumSaturatedRings(m))
desc.append(Chem.Lipinski.RingCount(m))
desc.append(Chem.rdMolDescriptors.CalcTPSA(m))
desc.append(Chem.rdMolDescriptors.CalcTPSA(m)) # J. Med. Chem. 43:3714-7, (2000)
desc.append(Chem.MolSurf.LabuteASA(m)) # J. Mol. Graph. Mod. 18:464-77 (2000)
descriptors.append(desc)
descriptors_df = pd.DataFrame(data = descriptors, columns = [x for x in range(len(descriptors[0]))])
if (outputs != None):
descriptors_df[output_name] = output
return descriptors_df
# Decomposes a list of molecules in smiles into cliques and returns a clique decompostion dataframe and the list of cliques
def get_clique_decomposition(mol_smiles, outputs= None, output_name = 'output'):
# Generating Cliques
vocab=cf.Vocabulary(mol_smiles)
size=len(vocab)
vocabl = list(vocab)
MolDict=cf.Vocab2Cat(vocab)
clustersTR=cf.Clusters(mol_smiles)
catTR=cf.Cluster2Cat(clustersTR,MolDict)
clique_decomposition=cf.Vectorize(catTR,size)
descriptors_df = pd.DataFrame(data=clique_decomposition, columns = [x for x in range(len(vocabl))])
if (outputs != None):
descriptors_df[output_name] = output
return descriptors_df, vocabl
def get_train_test_splits(descriptor_df, output,KFold):
# Generating train test splits
splits = {'xtrain':[],
'xtest':[],
'ytrain':[],
'ytest':[]}
for train_index, test_index in KFold.split(descriptor_df.iloc[:,:],output):
splits['xtrain'].append(descriptor_df.iloc[train_index,:])
splits['xtest'].append(descriptor_df.iloc[test_index,:])
splits['ytrain'].append(output.iloc[train_index])
splits['ytest'].append(output.iloc[test_index])
return splits
def get_average_history(history_list):
average_history = np.zeros(len(history_list[0]))
for kfold in history_list: # loop through KFolds
for epoch_index, epoch_hist in zip(range(len(kfold)), kfold): # loop through epochs
average_history[epoch_index] += epoch_hist
average_history = average_history/5
return average_history
def get_std_history(history_list):
std_history = np.zeros(len(history_list[0]))
for x in range(len(history_list[0])):
epoch_i_values = [] # list to store loss at epoch i across k folds
for fold in history_list: # Loop through kfold
epoch_i_values.append(fold[x])
std_history[x] = np.std(epoch_i_values)
return std_history
def work_up(GridSearchCVKerasResults):
train_mae_averages = []
train_mae_stds = []
train_mae_min = []
train_mse_averages = []
train_mse_stds = []
train_mse_min = []
test_mae_averages = []
test_mae_stds = []
test_mae_min = []
test_mse_averages = []
test_mse_min = []
test_mse_stds =[]
for row in GridSearchCVKerasResults.index:
train_mae_list = GridSearchCVKerasResults.loc[row,['train_mae_hist_1','train_mae_hist_2','train_mae_hist_3','train_mae_hist_4','train_mae_hist_5']]
train_mse_list = GridSearchCVKerasResults.loc[row,['train_mse_hist_1','train_mse_hist_2','train_mse_hist_3','train_mse_hist_4','train_mse_hist_5']]
test_mae_list = GridSearchCVKerasResults.loc[row,['test_mae_hist_1','test_mae_hist_2','test_mae_hist_3','test_mae_hist_4','test_mae_hist_5']]
test_mse_list = GridSearchCVKerasResults.loc[row,['test_mse_hist_1','test_mse_hist_2','test_mse_hist_3','test_mse_hist_4','test_mse_hist_5']]
train_mae_averages.append(get_average_history(train_mae_list))
train_mse_averages.append(get_average_history(train_mse_list))
test_mae_averages.append(get_average_history(test_mae_list))
test_mse_averages.append(get_average_history(test_mse_list))
train_mae_stds.append(get_std_history(train_mae_list))
train_mse_stds.append(get_std_history(train_mse_list))
test_mae_stds.append(get_std_history(test_mae_list))
test_mse_stds.append(get_std_history(test_mse_list))
for train_mae, train_mse, test_mae, test_mse in zip(train_mae_averages, train_mse_averages, test_mae_averages, test_mse_averages):
train_mae_min.append(min(train_mae))
train_mse_min.append(min(train_mse))
test_mae_min.append(min(test_mae))
test_mse_min.append(min(test_mse))
GridSearchCVKerasResults['train_mae_average'] = train_mae_averages
GridSearchCVKerasResults['train_mse_average'] = train_mse_averages
GridSearchCVKerasResults['test_mae_average'] = test_mae_averages
GridSearchCVKerasResults['test_mse_average'] = test_mse_averages
GridSearchCVKerasResults['train_mae_std'] = train_mae_stds
GridSearchCVKerasResults['train_mse_std'] = train_mse_stds
GridSearchCVKerasResults['test_mae_std'] = test_mae_stds
GridSearchCVKerasResults['test_mse_std'] = test_mse_stds
GridSearchCVKerasResults['train_mae_min'] = train_mae_min
GridSearchCVKerasResults['train_mse_min'] = train_mse_min
GridSearchCVKerasResults['test_mae_min'] = test_mae_min
GridSearchCVKerasResults['test_mse_min'] = test_mse_min
return GridSearchCVKerasResults
def get_best_model_index_mse(worked_up_results):
best_ind = worked_up_results.loc[worked_up_results['test_mse_min'] == min(worked_up_results['test_mse_min'])].index[0]
return best_ind
def refit_best_model(worked_up_results, splits, scaler,unscaled_outputs):
scaler.fit(np.array(unscaled_outputs).reshape(-1,1))
best_ind = get_best_model_index_mse(worked_up_results)
best_arch = worked_up_results['architecture'][best_ind]
best_acti = worked_up_results['activation'][best_ind]
best_opti = worked_up_results['optimiser'][best_ind]
df = pd.DataFrame(worked_up_results['test_mse_average'][best_ind])
best_epoch = df.loc[df[0] == min(df[0])].index[0]
tr_pred_list = []
te_pred_list = []
for xtr, xte, ytr, yte in zip(splits['xtrain'],splits['xtest'],
splits['ytrain'],splits['ytest']):
best_model = build_model(xtr, architecture=best_arch, activation=best_acti, optimiser=best_opti)
best_model.fit(xtr,ytr,validation_data = [xte,yte],epochs = best_epoch,verbose = 0)
tr_pred_list.append(best_model.predict(xtr))
te_pred_list.append(best_model.predict(xte))
tr_pred_inv_tr_list = []
te_pred_inv_tr_list = []
ytr_inv_tr_list = []
yte_inv_tr_list = []
for tr_pred, te_pred, ytr,yte in zip(tr_pred_list,te_pred_list,splits['ytrain'],splits['ytest']):
tr_pred_inv_tr_list.append(scaler.inverse_transform(tr_pred))
te_pred_inv_tr_list.append(scaler.inverse_transform(te_pred))
ytr_inv_tr_list.append(scaler.inverse_transform(ytr))
yte_inv_tr_list.append(scaler.inverse_transform(yte))
return tr_pred_inv_tr_list, te_pred_inv_tr_list, ytr_inv_tr_list, yte_inv_tr_list
def plot_results(worked_up_results,splits, scaler,unscaled_outputs,fig_title):
best_ind = get_best_model_index_mse(worked_up_results)
fig, ((ax1,ax2),(ax3,ax4),(ax5,ax6)) = plt.subplots(3,2,figsize=[12,16],dpi=200)
plt.suptitle(fig_title)
# PLotting Model Accuracies
ax1.bar([x for x in range(len(worked_up_results['test_mse_min']))],
worked_up_results['test_mse_min'],color='black',edgecolor='orange')
#ax1.set_ylim(min(worked_up_results['test_mse_min'])-0.01,max(worked_up_results['test_mse_min'])+0.01)
ax1.set_title('Model Performance')
ax1.set_ylabel('Mean Squared Error (scaled units)')
ax1.set_xlabel('Model Number')
# Plotting loss history
ax2.plot(worked_up_results['epoch'][best_ind],
worked_up_results['test_mse_average'][best_ind], label = 'Test Loss History')
ax2.plot(worked_up_results['epoch'][best_ind],
worked_up_results['train_mse_average'][best_ind], label = 'Train Loss History')
ax2.set_title('Best Model history')
ax2.set_ylabel('Mean Squared Error')
ax2.set_xlabel('Epoch')
# Plotting error bars train
ax3.plot(worked_up_results['epoch'][best_ind],
worked_up_results['train_mse_average'][best_ind], label = 'Train Loss History',color='k')
ax3.plot(worked_up_results['epoch'][best_ind],
worked_up_results['train_mse_average'][best_ind]+worked_up_results['train_mse_std'][best_ind],color='k')
ax3.plot(worked_up_results['epoch'][best_ind],
worked_up_results['train_mse_average'][best_ind]-worked_up_results['train_mse_std'][best_ind],color='k')
ax3.fill_between(worked_up_results['epoch'][best_ind], worked_up_results['train_mse_average'][best_ind]-worked_up_results['train_mse_std'][best_ind],
worked_up_results['train_mse_average'][best_ind]+worked_up_results['train_mse_std'][best_ind])
ax3.set_title('Best Model history Train with Errors')
ax3.set_ylabel('Mean Squared Error')
ax3.set_xlabel('Epoch')
# Plotting error bars train
ax4.plot(worked_up_results['epoch'][best_ind],
worked_up_results['test_mse_average'][best_ind], label = 'Test Loss History',color='k')
ax4.plot(worked_up_results['epoch'][best_ind],
worked_up_results['test_mse_average'][best_ind]+worked_up_results['test_mse_std'][best_ind],color='k')
ax4.plot(worked_up_results['epoch'][best_ind],
worked_up_results['test_mse_average'][best_ind]-worked_up_results['test_mse_std'][best_ind],color='k')
ax4.fill_between(worked_up_results['epoch'][best_ind], worked_up_results['test_mse_average'][best_ind]-worked_up_results['test_mse_std'][best_ind],
worked_up_results['test_mse_average'][best_ind]+worked_up_results['test_mse_std'][best_ind])
ax4.set_title('Best Model history Test with Errors')
ax4.set_ylabel('Mean Squared Error')
ax4.set_xlabel('Epoch')
# Plotting predicted vs experimental
tr_pred, te_pred, ytr, yte = refit_best_model(worked_up_results, splits, scaler, unscaled_outputs)
acc = np.linspace(min(ytr[0]),max(ytr[0]),1000)
ax5.plot(acc,acc)
ax5.scatter(ytr[0],tr_pred[0],label='Train Set')
ax5.scatter(yte[0],te_pred[0],label='Test Set')
ax5.set_ylabel('Predicted Value')
ax5.set_xlabel('Experimental Value')
# Plotting distribution of errors
train_errors = []
test_errors = []
for tr_pred, te_pred, ytr, yte in zip(tr_pred[0],te_pred[0],ytr[0],yte[0]):
train_errors.append(ytr-tr_pred)
test_errors.append(yte-te_pred)
train_errors = np.array(train_errors).reshape(-1)
test_errors = np.array(test_errors).reshape(-1)
#ax6.hist(train_errors)
#ax6.hist(test_errors)
sns.distplot(train_errors , color="skyblue", label="Train Errors")
sns.distplot(test_errors , color="red", label="Test Errors")
ax6.set_title('Distribution of prediction errors')
ax1.legend()
ax2.legend()
ax3.legend()
ax4.legend()
ax5.legend()
ax6.legend()
<file_sep>import pandas as pd
import numpy as np
from numpy import inf
from rdkit import Chem
from rdkit.Chem import Draw
from rdkit.Chem import AllChem
from rdkit.Chem import Descriptors
from mendeleev import element
import h5py
import collections
from scipy.spatial import distance
from scipy.spatial import distance_matrix
m = Chem.MolFromSmiles('CC(C)C1=NC(=CS1)CN(C)C(=O)NC(C(C)C)C(=O)NC(CC2=CC=CC=C2)CC(C(CC3=CC=CC=C3)NC(=O)OCC4=CN=CS4)')
class SymmetryVec:
def __init__(self, smile, Nrad, Nang, rcAng, rcRad):
self.smile = smile
# self.dist = h5py.File(distPath,'r')
# self.ang = h5py.File(angPath,'r')
# self.distMat = self.dist[smile][:]
# self.angMat = self.ang[smile][:]
self.Nrad = Nrad
self.Nang = Nang
self.rcRad = rcRad
self.rcAng = rcAng
self.coords = self.xyz_dict()
self.distMat = distance_matrix(self.coords,self.coords)
self.muListRad = self.muList(rcRad, Nrad)
self.muListAng = self.muList(rcAng, Nang)
self.etaRad = 1/(2 * np.square((rcRad - 1.5)/(Nrad -1)))
self.etaAng = 1/(2 * np.square((rcAng - 1.5)/(Nang -1)))
self.atomList = self.atomList()
self.weights = [element(atom).atomic_number for atom in self.atomList ]
self.radVector = self.wACSF_Rad()
self.angArray = self.makeAngleArray()
self.angVector =self.wACSF_Ang()
def unit_vector(self, vector):
np.seterr(divide='ignore')
return vector / np.linalg.norm(vector)
def angle_between(self, v1, v2):
v1_u = self.unit_vector(v1)
v2_u = self.unit_vector(v2)
return np.clip(np.dot(v1_u, v2_u), -1.0, 1.0)
def atomList(self):
m = Chem.MolFromSmiles(self.smile) # The molecule from smiles
mH = Chem.AddHs(m)
numAtoms = mH.GetNumAtoms()
atomList = []
for i in range(numAtoms):
atomList.append(mH.GetAtomWithIdx(i).GetSymbol())
return atomList
def muList(self, rc, N):
mu = [0.5]
for i in range(N-1):
mu.append(mu[i] + (rc - 1.5)/(N -1))
return mu
def xyz_dict(self):
list = []
m = Chem.MolFromSmiles(self.smile) # The molecule from smiles
mH = Chem.AddHs(m)# Adding Hydrogen
n = mH.GetNumAtoms()
AllChem.EmbedMolecule(mH) # Initial coordinates of a molecule
AllChem.UFFOptimizeMolecule(mH) # Optimise molecule?
for i in range(n) :# For each atom
coords = []
pos = mH.GetConformer().GetAtomPosition(i) # Get position coordinates
coords.append(pos.x)
coords.append(pos.y)
coords.append(pos.z)
list.append(coords)
return np.array(list)
def wACSF_Rad(self):
list = []
for mu in self.muListRad:
fvals = 0.5 * (np.cos((self.distMat * np.pi)/self.rcRad) + 1)
mask = np.where((self.distMat < self.rcRad) & (self.distMat > 0), 1, 0 )
vals = np.exp(-self.etaRad * (self.distMat-mu)**2)
unmasked_wACSF = fvals * (vals.T * self.weights).T
masked_wACSF = unmasked_wACSF * mask
final = np.sum(masked_wACSF, axis = 0)
list.append(final)
return (np.array(list).T)
def unit_vector(self, vector):
return vector / np.linalg.norm(vector)
def angle_between(self, v1, v2):
v1_u = self.unit_vector(v1)
v2_u = self.unit_vector(v2)
return np.clip(np.dot(v1_u, v2_u), -1.0, 1.0)
def makeAngleArray(self):
coords = self.coords
n = len(coords)
array = np.zeros([n,n,n])
for i in range(n):
for j in range(n):
for k in range(n):
ij = coords[j] - coords[i]
ik = coords[k] - coords[i]
cosTheta = self.angle_between(ij,ik)
# theta = arccos(clip(cosTheta, -1, 1))
array[i,j,k] = cosTheta
return np.nan_to_num(array)
def wACSF_Ang(self, zeta = 1): # get distMat, atomList from distanceMatrix function. i is int for atom in atomList. angleArray from makeAngleArray function.
lam = [1, -1]
list = []
for l in lam:
for mu in self.muListAng:
expMats = np.exp(-self.etaAng*(self.distMat-mu)**2)
exp3d = np.einsum('ij,ik,jk->ijk',expMats,expMats,expMats)
fMats = 0.5*(np.cos((self.distMat * np.pi)/self.rcAng)+1)
f3d = np.einsum('ij,ik,jk->ijk',fMats,fMats,fMats)
maskMats = np.where((self.distMat < self.rcAng) & (self.distMat > 0), 1, 0 )
mask3d = np.einsum('ij,ik,jk->ijk',maskMats,maskMats,maskMats)
angArrayMod = (1 + (self.angArray * l))**zeta
weights3d = np.einsum('i,j->ij', self.weights,self.weights)
finWeighted = np.einsum('ijk,ijk,ijk->ijk',exp3d,f3d,angArrayMod)
finMasked = np.einsum('ijk,ijk->ijk', finWeighted, mask3d)
wACSFvec = np.einsum('jk,ijk->i', weights3d, finMasked)
list.append(wACSFvec)
return np.array(list).T
class histToDf:
def __init__(self,radVector, angVector, Nrad, Nang, bins):
self.angArray = angVector
self.radArray = radVector
self.Nrad = Nrad
self.Nang = Nang * 2
self.bins = bins
self.minRad, self.maxRad, self.minAng, self.maxAng = self.minMax()
def minMax(self):
minRad, maxRad = np.amin(self.radArray), np.amax(self.radArray)
minAng, maxAng = np.amin(self.angArray), np.amax(self.angArray)
return minRad, maxRad, minAng, maxAng
def binningList(self):
list = []
for i in range(self.Nrad):
data = np.histogram(self.radArray[:,i],bins = self.bins,range =(self.minRad,self.maxRad))[0]
list.extend(data)
for i in range(self.Nang):
data = np.histogram(self.angArray[:,i] ,bins = self.bins,range =(self.minAng,self.maxAng))[0]
list.extend(data)
return np.array(list)
def histWACSF(smilesList, Nrad, Nang, rcRad, rcAng, bins):
numCols = (2*Nang +Nrad) * bins
array = np.empty((0,(numCols)))
for smile in smilesList:
data = SymmetryVec(smile, Nrad, Nang, rcAng, rcRad)
ang = data.angVector
rad = data.radVector
temp = histToDf(rad, ang, Nrad, Nang, bins)
row = temp.binningList().reshape(1,numCols)
array = np.append(array, row, axis=0)
return(array)
def makeDF(dataFrameEmpty, histWACSF, Nrad, Nang, bins):
for i in range(Nrad):
for j in range(bins):
dataFrameEmpty['wACSF_Rad{}_{}'.format(i,j)]=0
for i in range(Nang * 2):
for j in range(bins):
dataFrameEmpty['wACSF_Ang{}_{}'.format(i,j)]=0
dataFrameEmpty.loc[:,'wACSF_Rad0_0':] = histWACSF
return dataFrameEmpty
<file_sep># MSDE_Sosso_alpha
The computational workflow used in <MSDE paper> is contained in the Jupyter Notebook: MSDE_Sosso_alpha.ipynb
An Anaconda environment file (generated on a machine running Linux Centos 7) listing the necessary dependencies can be
found in the ./PYTHON directory as MSDE_Sosso_alpha.yml. The following additional packages can be installed via pip:
- tensorflow
- keras
- mendeleev
- gpy
The latest (as of August 2019) version of pandas (pandas-0.24.2) is also needed, e.g.
pip install --upgrade pandas
| e097afde1de097cb983f18ce7c69d9fbd8a76791 | [
"Markdown",
"Python"
] | 6 | Python | arnabmukho/MSDE_Sosso_alpha | 3bb996436e0d6b10ac6b4f0289ae1871a57ef20b | 8acc9a42ba8e19ab009f275f9a9f4ab7b1513ecd | |
refs/heads/master | <repo_name>Baranax/Python-Practice<file_sep>/notes.py
"""
Name :<NAME>
Title :index.py
Version :1.0.1
Copyright :ALL RIGHTS RESERVED
Description:Main Python module for project
"""
# function definitions
def user_list():
print('--Main menu--')
print('"load" - opens a previous stack (if applicable)')
print('"add" - adds a note to the notes stack')
print('"print" - prints all notes currently in stack')
print('"insert" - inserts a note at selected location')
print('"remove" - removes a note at selected location')
print('"save" - saves notes to persistent file')
print('"export" - exports notes to xml file')
print('"quit" - exits the program safely')
def user_add():
note_input = str(input('Type "single" or "multiple": '))
if note_input == 'single':
new_note = str(input('Note to be added: '))
userNotes.append(new_note)
print('Note added successfully!')
elif note_input == 'multiple':
while note_input != 'DONE':
new_note = str(input('Note to be added (type DONE to finish adding notes): '))
userNotes.append(new_note)
print('Note added successfully!')
if new_note == 'DONE':
note_input = new_note
def user_print():
print('Current list of notes:')
print(*userNotes, sep='\n')
def user_insert():
note_location = int(input('Location of new note: '))
new_note = str(input('Note to be added: '))
userNotes.insert(note_location, new_note)
print('Note added successfully at %d!' % note_location)
def user_remove():
note_location = int(input('Location of note: '))
userNotes.pop(note_location)
print('Note deleted successfully at %d!' % note_location)
def user_save():
print('WARNING! MODULE UNDER CONSTRUCTION!')
index = open('index.txt', 'w')
index.writelines("%s\n" % item for item in userNotes)
# variable declaration
userNotes = []
# init program
print('Program is now running...')
print('\nNotes program - type "list" for a list of commands')
userInput = str(input('Option: '))
while userInput != 'quit':
if userInput == 'list':
user_list()
elif userInput == 'load':
print('Module not yet complete!')
elif userInput == 'add':
user_add()
elif userInput == 'print':
user_print()
elif userInput == 'insert':
user_insert()
elif userInput == 'remove':
user_remove()
elif userInput == 'save':
user_save()
elif userInput == 'export':
print('Module not yet implemented!')
else:
print('INVALID OPTION!')
userInput = str(input('Option: '))
print('Good Bye!')
<file_sep>/AreaCalculator.py
from math import pi
from time import sleep
from datetime import datetime
now = datetime.now()
print "Calculator is now running..."
print "Current time:%s/%s/%s/%s:%s" % (now.month, now.day, now.year, now.hour, now.minute)
sleep(1)
hint = "Don't forget to include the correct unit!"
option = raw_input("Enter C for Circle or T for Triangle:")
option = option.upper()
#check if shape is circle or triangle and evaluate area
if option == 'C':
radius = float(raw_input("Input radius: "))
area = pi * (radius**2)
print "The pie is baking..."
sleep(1)
print ("Area: %.2f. \n%s" % (area, hint))
elif option == 'T':
base = float(raw_input("Input base: "))
height = float(raw_input("Input height: "))
area = base * height * (.5)
print "Uni Bi Tri..."
sleep(1)
print ("Area: %.2f \n%s" % (area, hint))
else:
print "Input incorrect. Program will now Exit..."
<file_sep>/nameandage.py
#A simple program to determine someone's name and age and then calculate when they will become 100 years old
print "Name and Age Calculator is now running"
name = "What is your name: "
age = "How old are you (in years): "
year = str((2016 - age) + 100)
print(name + " will be 100 years old in the year " + year)
<file_sep>/README.md
# Python-Practice
# This is how we do things around here
<file_sep>/Madlibs.py
## This program was made to take a list of adjectives from the user and add it to the pre-defined story below
print "Mad Libs is now running"
char_name = raw_input("Please type a name ")
adj1 = raw_input("adjective 1 ")
adj2 = raw_input("adjective 2 ")
adj3 = raw_input("adjective 3 ")
verb1 = raw_input("verb 1 ")
verb2 = raw_input("verb 2 ")
verb3 = raw_input("verb 3 ")
noun1 = raw_input("noun 1 ")
noun2 = raw_input("noun 2 ")
noun3 = raw_input("noun 3 ")
noun4 = raw_input("noun 4 ")
animal = raw_input("animal ")
food = raw_input("food ")
fruit = raw_input("fruit ")
number = raw_input("number ")
superhero_name = raw_input("Superhero Name ")
country = raw_input("country ")
dessert = raw_input("dessert ")
year = raw_input("year ")
#The template for the story
STORY = "This morning I woke up and felt %s because %s was going to finally %s over the big %s %s. On the other side of the %s were many %ss protesting to keep %s in stores. The crowd began to %s to the rythym of the %s, which made all of the %ss very %s. %s tried to %s into the sewers and found %s rats. Needing help, %s quickly called %s. %s appeared and saved %s by flying to %s and dropping %s into a puddle of %s. %s then fell asleep and woke up in the year %s, in a world where %ss ruled the world."
print STORY % (adj1, char_name, verb1, adj2, noun1, noun2, animal, food, verb2, noun3, fruit, adj3, char_name, verb3, number, char_name, superhero_name, superhero_name, char_name, country, char_name, dessert, char_name, year, noun4)
| 46a2bb9d79ff9c07b8831bfbb45a2dba2b59e821 | [
"Markdown",
"Python"
] | 5 | Python | Baranax/Python-Practice | e7d03415d1ab0ebfc86d1bc5b7ef32c9d4621ed1 | dc55b448ab1104a43ce6befc2940a9d659e2ff9d | |
refs/heads/master | <repo_name>pf2019g4/utn-simulador-ui<file_sep>/src/app/premios-detail/premios-detail.component.ts
import { Component, OnInit } from '@angular/core';
import { ActivatedRoute } from '@angular/router';
import { EscenariosService } from '../escenarios.service';
import { MessagesService } from '../messages.service';
import { UsuarioService } from '../usuario.service';
@Component({
selector: 'app-premios-detail',
templateUrl: './premios-detail.component.html',
styleUrls: ['./premios-detail.component.css']
})
export class PremiosDetailComponent implements OnInit {
escenario;
curso;
constructor(private route: ActivatedRoute,
private escenariosService: EscenariosService,
private usuarioService: UsuarioService,
private messageService: MessagesService) { }
ngOnInit() {
const escenarioId = Number(this.route.snapshot.paramMap.get('escenarioId'));
this.curso = this.usuarioService.usuario.curso;
this.getEscenario(escenarioId);
}
getEscenario(escenarioId) {
this.escenariosService.getEscenario(escenarioId).subscribe(escenario => {
this.escenario = escenario
})
}
}
<file_sep>/src/app/app-routing.module.ts
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
import { PremiosComponent } from './premios/premios.component';
import { HomeComponent } from './home/home.component';
import { LoginComponent } from './login/login.component';
import { DecisionesComponent } from './decisiones/decisiones.component';
import { DecisionDetalleComponent } from './decision-detalle/decision-detalle.component';
import { FlujoFondosComponent } from './flujo-fondos/flujo-fondos.component';
import { TomaDecisionesComponent } from './toma-decisiones/toma-decisiones.component';
import { ResultadosComponent } from './resultados/resultados.component';
import { ResultadosAdminComponent } from './resultados-admin/resultados-admin.component';
import { EscenariosComponent } from './escenarios/escenarios.component';
import { EscenarioDetalleComponent } from './escenario-detalle/escenario-detalle.component';
import { SimulacionesComponent } from './simulaciones/simulaciones.component';
import { ProveedorDetailComponent } from './proveedor-detail/proveedor-detail.component';
import { EstadoJuegosComponent } from './estado-juegos/estado-juegos.component';
import { CursosComponent } from './cursos/cursos.component';
import { EstadoJuegoDetailComponent } from './estado-juego-detail/estado-juego-detail.component';
import { SimulacionEntregadaComponent } from './simulacion-entregada/simulacion-entregada.component';
import { PremiosDetailComponent } from './premios-detail/premios-detail.component';
const routes: Routes = [
{ path: '', redirectTo: '/login', pathMatch: 'full' },
{ path: 'login', component: LoginComponent },
{ path: 'home', redirectTo: 'simulaciones' },
{
path: 'simulaciones', component: HomeComponent,
children: [{ path: '', component: SimulacionesComponent, pathMatch: 'full' }]
},
{
path: 'simulacion-entregada', component: HomeComponent,
children: [{ path: '', component: SimulacionEntregadaComponent, pathMatch: 'full' }]
},
{
path: 'simulaciones/escenario/:escenarioId/resultados', component: HomeComponent,
children: [{ path: '', component: ResultadosComponent, pathMatch: 'full' }]
},
{
path: 'simulaciones/escenario/:escenarioId/resultados/curso/:cursoId/usuario/:usuarioId', component: HomeComponent,
children: [{ path: '', component: ResultadosAdminComponent, pathMatch: 'full' }]
},
{
path: 'premios', component: HomeComponent,
children: [{ path: '', component: PremiosComponent, pathMatch: 'full' }]
},
{
path: 'premios/escenario/:escenarioId', component: HomeComponent,
children: [{ path: '', component: PremiosDetailComponent, pathMatch: 'full' }]
},
{
path: 'escenarios', component: HomeComponent,
children: [{ path: '', component: EscenariosComponent, pathMatch: 'full' }]
},
{
path: 'cursos', component: HomeComponent,
children: [{ path: '', component: CursosComponent, pathMatch: 'full' }]
},
{
path: 'estado-juegos', component: HomeComponent,
children: [{ path: '', component: EstadoJuegosComponent, pathMatch: 'full' }]
},
{
path: 'simulaciones/escenario/:escenarioId', component: HomeComponent,
children: [{ path: '', component: TomaDecisionesComponent, pathMatch: 'full' }]
},
{
path: 'estado-juego/curso/:cursoId/escenario/:escenarioId', component: HomeComponent,
children: [{ path: '', component: EstadoJuegoDetailComponent, pathMatch: 'full' }]
},
{ path: 'escenarios/:escenarioId/decisiones/new', component: DecisionDetalleComponent, pathMatch: 'full' },
{ path: 'escenarios/:escenarioId/decisiones/:id', component: DecisionDetalleComponent, pathMatch: 'full' },
{ path: 'escenarios/:escenarioId/proveedores/new', component: ProveedorDetailComponent, pathMatch: 'full' },
{ path: 'escenarios/:escenarioId/proveedores/:id', component: ProveedorDetailComponent, pathMatch: 'full' },
{ path: 'escenarios/new', component: EscenarioDetalleComponent, pathMatch: 'full' },
{ path: 'escenarios/:id', component: EscenarioDetalleComponent }
];
@NgModule({
imports: [RouterModule.forRoot(routes, { useHash: true })],
exports: [RouterModule]
})
export class AppRoutingModule { }
<file_sep>/src/app/proveedor-detail/proveedor-detail.component.ts
import { Component, OnInit } from '@angular/core';
import { Validators, FormControl, FormGroup } from '@angular/forms';
import { ProveedoresService } from '../proveedores.service';
import { ActivatedRoute, Router } from '@angular/router';
import { MessagesService } from '../messages.service';
import { EscenariosService } from '../escenarios.service';
@Component({
selector: 'app-proveedor-detail',
templateUrl: './proveedor-detail.component.html',
styleUrls: ['./proveedor-detail.component.css']
})
export class ProveedorDetailComponent implements OnInit {
proveedor: any = {
escenarioId: null,
nombre: "",
variacionCostoVariable: 0,
variacionCalidad: 0,
modalidadPago: [{
porcentaje: 0,
offsetPeriodo: 0
}, {
porcentaje: 0,
offsetPeriodo: 1
}, {
porcentaje: 0,
offsetPeriodo: 2
}, {
porcentaje: 0,
offsetPeriodo: 3
}]
};
nombre = new FormControl('', [Validators.required]);
variacionCostoVariable = new FormControl('', [Validators.required]);
variacionCalidad = new FormControl('', [Validators.required]);
proveedorForm: FormGroup = new FormGroup({
nombre: this.nombre,
variacionCostoVariable: this.variacionCostoVariable,
variacionCalidad: this.variacionCalidad
});
constructor(private proveedoresService: ProveedoresService,
private route: ActivatedRoute,
private router: Router,
private messageService: MessagesService,
private escenariosService: EscenariosService) { }
ngOnInit() {
var esenarioId = Number(this.route.snapshot.paramMap.get('escenarioId'));
var id = Number(this.route.snapshot.paramMap.get('id'));
if (id) {
this.escenariosService.getProveedor(esenarioId, id).subscribe(p => {
p.modalidadPago = this.proveedor.modalidadPago.map(mpt => {
return { ...mpt, ...p.modalidadPago.find(mp => mp.offsetPeriodo === mpt.offsetPeriodo) };
});
this.proveedor = p;
this.proveedor.escenarioId = esenarioId;
});
} else {
this.proveedor.escenarioId = esenarioId;
}
}
save() {
if (this.proveedorForm.valid) {
if (this.inputsValidos()) {
this.proveedoresService[this.proveedor.id ? 'modifyProveedor' : 'createProveedor'](this.proveedor)
.subscribe(_ => {
this.messageService.openSnackBar("Proveedor modificado");
this.router.navigate([`/escenarios/${this.proveedor.escenarioId}`])
})
}
} else {
this.messageService.openSnackBarDatosIngresados()
}
}
inputsValidos() {
//Validar totales de modalidad de pago
var modalidadPagoPorcentajeTotal = this.proveedor.modalidadPago.filter(elem => elem.porcentaje > 0)
.reduce((acum, elem) => acum + elem.porcentaje, 0)
if (modalidadPagoPorcentajeTotal !== 100) {
this.messageService.openSnackBar("Los porcentajes en la modalidad de pago deben sumar 100%!");
return false;
}
return true;
}
}
<file_sep>/src/app/decision-detalle/decision-detalle.component.ts
import { Component, OnInit } from '@angular/core';
import { Validators, FormControl, FormGroup } from '@angular/forms';
import { ActivatedRoute, Router } from '@angular/router';
import { animate, state, style, transition, trigger } from '@angular/animations';
import { MatDialog } from '@angular/material';
import { OpcionDialogComponent } from './opciones/opcion-dialog.component';
import { DecisionesService } from '../decisiones.service';
import { ConsecuenciaDialogComponent } from './consecuencias/consecuencia-dialog.component';
import { MessagesService } from '../messages.service';
import { EscenariosService } from '../escenarios.service';
import { ProyectoService } from '../proyecto.service';
@Component({
selector: 'app-decision-detalle',
templateUrl: './decision-detalle.component.html',
styleUrls: ['./decision-detalle.component.css'],
animations: [
trigger('detailExpand', [
state('collapsed', style({ height: '0px', minHeight: '0' })),
state('expanded', style({ height: '*' })),
transition('expanded <=> collapsed', animate('225ms cubic-bezier(0.4, 0.0, 0.2, 1)')),
]),
]
})
export class DecisionDetalleComponent implements OnInit {
tipoCuentas;
decision: any = {
escenarioId: null,
descripcion: "",
opciones: []
};
descripcion = new FormControl('', [Validators.required]);
decisionForm: FormGroup = new FormGroup({
descripcion: this.descripcion
});
displayedColumns: string[] = ['descripcion', 'edit'];
constructor(private decisionesService: DecisionesService,
private route: ActivatedRoute,
private dialog: MatDialog,
private router: Router,
private messageService: MessagesService,
private escenariosService: EscenariosService,
private proyectoService: ProyectoService) { }
ngOnInit() {
var esenarioId = Number(this.route.snapshot.paramMap.get('escenarioId'));
var id = Number(this.route.snapshot.paramMap.get('id'));
if (id) {
this.escenariosService.getDecision(esenarioId, id).subscribe(d => {
this.decision = d;
this.decision.escenarioId = esenarioId;
});
} else {
this.decision.escenarioId = esenarioId;
}
this.getTipoCuentas();
}
getTipoCuentas() {
this.proyectoService.getTipoCuentas().subscribe(tipoCuentas => this.tipoCuentas = tipoCuentas)
}
tipoCuentaDescripcion(tipoCuenta) {
if (this.tipoCuentas) {
const tipoCuentaObject = this.tipoCuentas.find(tc => tc.key === tipoCuenta)
return tipoCuentaObject && tipoCuentaObject.descripcion;
}
}
addOpcion() {
const dialogRef = this.dialog.open(OpcionDialogComponent, {
width: '400px',
data: {
decisionId: this.decision.id,
descripcion: "",
variacionCostoFijo: 0,
variacionCostoVariable: 0,
variacionProduccion: 0,
variacionCalidad: 0,
variacionPublicidad: 0,
variacionCantidadVendedores: 0,
consecuencias: []
}
});
var that = this;
dialogRef.afterClosed().subscribe(updatedOpcion => {
if (updatedOpcion) {
that.decision.opciones.push(updatedOpcion);
that.refreshDecisiones();
}
});
}
editOpcion(opcion) {
const dialogRef = this.dialog.open(OpcionDialogComponent, {
width: '400px',
data: opcion
});
var that = this;
dialogRef.afterClosed().subscribe(updatedOpcion => {
if (updatedOpcion) {
var index = that.decision.opciones.indexOf(opcion);
if (index !== -1) {
that.decision.opciones[index] = updatedOpcion;
}
that.refreshDecisiones();
}
});
}
removeOpcion(opcion) {
var index = this.decision.opciones.indexOf(opcion);
this.decision.opciones.splice(index, 1)
this.refreshDecisiones();
}
addConsecuencia(opcion) {
const dialogRef = this.dialog.open(ConsecuenciaDialogComponent, {
width: '400px',
data: {
opcionId: opcion.id,
descripcion: "",
monto: null,
periodoInicio: 0,
cantidadPeriodos: 1,
tipoCuenta: "ECONOMICO"
}
});
dialogRef.afterClosed().subscribe(updatedConsecuencia => {
if (updatedConsecuencia) {
opcion.consecuencias.push(updatedConsecuencia);
this.refreshConsecuencias(opcion);
}
});
}
editConsecuencia(opcion, consecuencia) {
const dialogRef = this.dialog.open(ConsecuenciaDialogComponent, {
width: '400px',
data: consecuencia
});
dialogRef.afterClosed().subscribe(updatedConsecuencia => {
if (updatedConsecuencia) {
var index = opcion.consecuencias.indexOf(consecuencia);
if (index !== -1) {
opcion.consecuencias[index] = updatedConsecuencia;
}
this.refreshConsecuencias(opcion);
}
});
}
removeConsecuencia(opcion, consecuencia) {
var index = opcion.consecuencias.indexOf(consecuencia);
opcion.consecuencias.splice(index, 1);
this.refreshConsecuencias(opcion);
}
refreshDecisiones() {
this.decision.opciones = this.decision.opciones.slice(0);
}
refreshConsecuencias(opcion) {
opcion.consecuencias = opcion.consecuencias.slice(0);
}
save() {
if (this.decisionForm.valid) {
this.decisionesService[this.decision.id ? 'modifyDecision' : 'createDecision'](this.decision)
.subscribe(_ => {
this.messageService.openSnackBar("Decision modificada");
this.router.navigate([`/escenarios/${this.decision.escenarioId}`])
})
} else {
this.messageService.openSnackBarDatosIngresados()
}
}
}
<file_sep>/src/app/escenarios.service.ts
import { Injectable } from '@angular/core';
import { HttpClient, HttpErrorResponse } from '@angular/common/http';
import { Observable, of } from 'rxjs';
import { environment } from '../environments/environment';
import { catchError, map } from 'rxjs/operators';
import { MessagesService } from './messages.service';
@Injectable({
providedIn: 'root'
})
export class EscenariosService {
getCursosPorEscenario(id: any) {
throw new Error("Method not implemented.");
}
constructor(private http: HttpClient, private messageService: MessagesService) { }
getEscenariosParaUsuario(idUsuario) {
return this.http.get(`${environment.proyectoServiceHost}/api/escenarios/usuario/${idUsuario}`)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
getEscenarios(): Observable<any> {
return this.http.get(`${environment.proyectoServiceHost}/api/escenarios`)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
getEscenario(id): Observable<any> {
return this.http.get(`${environment.proyectoServiceHost}/api/escenarios/${id}`)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
modifyEscenario(escenario) {
return this.http.put(`${environment.proyectoServiceHost}/api/escenarios/${escenario.id}`, escenario)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
duplicateEscenario(escenario) {
return this.http.post(`${environment.proyectoServiceHost}/api/escenarios/${escenario.id}`, escenario)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
createEscenario(escenario) {
delete escenario.id;
return this.http.post(`${environment.proyectoServiceHost}/api/escenarios`, escenario)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
deleteEscenario(id): Observable<any> {
return this.http.delete(`${environment.proyectoServiceHost}/api/escenarios/${id}`)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
getDecisiones(idEscenario): Observable<any> {
return this.http.get(`${environment.proyectoServiceHost}/api/escenario/${idEscenario}/decisiones`)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
getProveedores(idEscenario): Observable<any> {
return this.http.get(`${environment.proyectoServiceHost}/api/escenario/${idEscenario}/proveedores`)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
getDecision(idEscenario, idDecision) {
return this.http.get(`${environment.proyectoServiceHost}/api/escenario/${idEscenario}/decisiones`)
.pipe(map((decisiones: Array<any>) => {
return decisiones.find(d => d.id === idDecision);
}));
}
getProveedor(idEscenario, idProveedor) {
return this.http.get(`${environment.proyectoServiceHost}/api/escenario/${idEscenario}/proveedores`)
.pipe(map((proveedores: Array<any>) => {
return proveedores.find(p => p.id === idProveedor);
}));
}
getFinanciaciones(idEscenario): Observable<any> {
return this.http.get(`${environment.proyectoServiceHost}/api/escenario/${idEscenario}/financiacion`)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
getCursosEscenario(idEscenario) {
return this.http.get(`${environment.proyectoServiceHost}/api/escenarios/${idEscenario}/cursos`)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
updateCursosEscenario(idEscenario, cursos) {
return this.http.put(`${environment.proyectoServiceHost}/api/escenarios/${idEscenario}/cursos`, cursos)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
getDetalleEscenarioUsuariosPorCurso(idEscenario, idCurso) {
return this.http.get(`${environment.proyectoServiceHost}/api/escenarios/${idEscenario}/cursos/${idCurso}`)
.pipe(map( (escenarioCurso:any) => {
escenarioCurso.jugadores.sort((a,b) => Number(a.puntaje) < Number(b.puntaje) ? 1 : -1 )
return escenarioCurso;
}),catchError(this.messageService.catchError.bind(this.messageService)));
}
getConfiguracionMercado(escenarioId) {
return this.http.get(`${environment.proyectoServiceHost}/api/escenario/${escenarioId}/configuracionMercado`)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)),
map(configuracionesMercado => {
var ponderacionesMercado = {
precioDesde: [],
modalidadCobro: [],
publicidadDesde: [],
calidadDesde: [],
vendedoresDesde: []
}
configuracionesMercado.ponderacionesMercado.forEach(pm => {
ponderacionesMercado[{
"PRECIO_DESDE": "precioDesde",
"MODALIDAD_DE_COBRO": "modalidadCobro",
"PUBLICIDAD_DESDE": "publicidadDesde",
"CALIDAD_DESDE": "calidadDesde",
"VENDEDORES_DESDE": "vendedoresDesde",
}[pm.concepto]].push(pm)
})
configuracionesMercado.ponderacionesMercado = ponderacionesMercado;
return configuracionesMercado;
}));
}
postConfiguracionesMercado(configuracionesMercado) {
const escenarioId = configuracionesMercado.restriccionPrecio.escenarioId,
configuracionesMercadoForPost = JSON.parse(JSON.stringify(configuracionesMercado));
configuracionesMercadoForPost.ponderacionesMercado = [
...configuracionesMercadoForPost.ponderacionesMercado.precioDesde,
...configuracionesMercadoForPost.ponderacionesMercado.modalidadCobro,
...configuracionesMercadoForPost.ponderacionesMercado.publicidadDesde,
...configuracionesMercadoForPost.ponderacionesMercado.calidadDesde,
...configuracionesMercadoForPost.ponderacionesMercado.vendedoresDesde
]
return this.http.post(`${environment.proyectoServiceHost}/api/escenario/${escenarioId}/configuracionMercado`, configuracionesMercadoForPost)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
simularMercado(escenarioId, cursoId) {
return this.http.post(`${environment.proyectoServiceHost}/api/escenario/${escenarioId}/curso/${cursoId}/simular-mercado`, {})
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
getPuntajes(escenarioId) {
return this.http.get(`${environment.proyectoServiceHost}/api/escenario/${escenarioId}/puntajeEscenario`)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
postPuntajes(escenarioId, puntajes) {
puntajes.escenarioId = escenarioId;
return this.http.post(`${environment.proyectoServiceHost}/api/escenario/${escenarioId}/puntajeEscenario`, puntajes)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
getProyectosByEscenario(escenarioId) {
return this.http.get(`${environment.proyectoServiceHost}/api/proyectosPorEscenario/${escenarioId}`)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
}
<file_sep>/src/app/messages.service.ts
import { Injectable, Component } from '@angular/core';
import { MatSnackBar } from '@angular/material';
import { throwError } from 'rxjs';
@Injectable({
providedIn: 'root'
})
export class MessagesService {
constructor(private _snackBar: MatSnackBar) { }
openSnackBar(message) {
this._snackBar.open(message, "OK", {
duration: 2000,
});
}
catchError(err) {
this.openSnackBar(err.error? err.error.message : "Error desconocido");
return throwError(err);
}
openSnackBarDatosIngresados(){
this._snackBar.open("Revise los datos ingresados", "OK", {
duration: 2000,
});
}
}
<file_sep>/src/app/cursos/curso-dialog/curso-dialog.component.ts
import { Component, OnInit, Inject } from '@angular/core';
import { MatDialogRef, MAT_DIALOG_DATA } from '@angular/material';
import { FormControl, Validators, FormGroup } from '@angular/forms';
import { MessagesService } from '../../messages.service';
import { Router } from '@angular/router';
import { CursosService } from '../../cursos.service';
@Component({
selector: 'curso-dialog',
templateUrl: './curso-dialog.component.html',
styleUrls: ['./curso-dialog.component.css']
})
export class CursoDialogComponent implements OnInit {
curso;
nombre = new FormControl('', [Validators.required])
clave = new FormControl('', [Validators.required])
cursoForm: FormGroup = new FormGroup({
nombre: this.nombre,
clave: this.clave
});
constructor( public dialogRef: MatDialogRef<CursoDialogComponent>,
@Inject(MAT_DIALOG_DATA) public data, private cursosService: CursosService,
private messageService: MessagesService) { }
ngOnInit() {
this.curso = JSON.parse(JSON.stringify(this.data));
}
cancel(){
this.dialogRef.close();
}
save() {
if (this.cursoForm.valid) {
let cursoSend = {id: this.curso.id, nombre: this.curso.nombre, clave: btoa(this.curso.clave)};
this.cursosService[this.curso.id ? 'modifyCurso' : 'createCurso'](cursoSend)
.subscribe(_ => {
this.messageService.openSnackBar("Curso modificado");
this.dialogRef.close();
})
}else {
this.messageService.openSnackBarDatosIngresados()
}
}
}
<file_sep>/src/app/usuario.service.ts
import { Injectable, Component } from '@angular/core';
import { environment } from '../environments/environment';
import { HttpClient, HttpErrorResponse } from '@angular/common/http';
import { Observable, of } from 'rxjs';
import { map, catchError, tap } from 'rxjs/operators';
import { MessagesService } from './messages.service';
@Injectable()
export class UsuarioService {
private _usuario;
constructor(private http: HttpClient,
private messageService: MessagesService) { }
get usuario() {
return this._usuario;
}
getUsuario(usuario): Observable<any> {
return this.http.post(`${environment.proyectoServiceHost}/api/usuario`,usuario)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)),
tap( usuario => this._usuario = usuario ));
}
getProyecto(idEscenario, idUsuario): Observable<any> {
return this.http.get(`${environment.proyectoServiceHost}/api/escenario/${idEscenario}/usuario/${idUsuario}/proyecto`)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
matricular(usuario,curso){
return this.http.post(`${environment.proyectoServiceHost}/api/usuario/${usuario.id}/matricular`, curso)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
}
<file_sep>/src/app/presupuesto-economico/presupuesto-economico.component.ts
import { Component, OnInit, Input } from '@angular/core';
import { ProyectoService } from '../proyecto.service';
import { ActivatedRoute } from '@angular/router';
import { UsuarioService } from '../usuario.service';
@Component({
selector: 'app-presupuesto-economico',
templateUrl: './presupuesto-economico.component.html',
styleUrls: ['./presupuesto-economico.component.css']
})
export class PresupuestoEconomicoComponent implements OnInit {
@Input() proyecto: any;
@Input() forecast: boolean;
presupuestoEconomico;
periodos;
escenario;
constructor(private proyectoService: ProyectoService) { }
ngOnInit() {
this.getPeriodos(this.proyecto.id);
this.getPresupuestoEconomico(this.proyecto.id);
this.escenario = this.proyecto.escenario;
}
getPresupuestoEconomico(proyectoId) {
this.proyectoService.getPresupuestoEconomico(proyectoId,this.forecast).subscribe(pe => {
this.presupuestoEconomico = pe;
})
}
getPeriodos(proyectoId) {
this.proyectoService.getPeriodoActual(proyectoId, this.forecast).subscribe(periodoActual => {
this.periodos = [...Array(periodoActual).keys(), periodoActual];
})
}
getCuentaPeriodo(cuentasPeriodo, periodo, defaultCero = true, signoInvertido = false) {
var periodo = cuentasPeriodo && cuentasPeriodo.find(cuentaPeriodo => cuentaPeriodo.periodo === periodo)
var montoCrudo = periodo ? periodo.monto : (defaultCero ? 0 : "");
montoCrudo = signoInvertido ? -montoCrudo : montoCrudo;
if (montoCrudo < 0) {
return "(" + Math.abs(montoCrudo).toLocaleString() + ")";
} else if (montoCrudo === 0) {
return montoCrudo;
} else {
return montoCrudo.toLocaleString();
}
}
getTotalCuentaPeriodo(cuentasPeriodo, signoInvertido = false) {
var acumulador = 0;
if (cuentasPeriodo) {
cuentasPeriodo.map(cp => acumulador = acumulador + cp.monto);
acumulador = signoInvertido ? -acumulador : acumulador;
if (acumulador < 0) {
return "(" + Math.abs(acumulador).toLocaleString() + ")";
} else if (acumulador === 0) {
return acumulador;
} else {
return acumulador.toLocaleString();
}
}
}
}
<file_sep>/src/app/financiacion.service.ts
import { Injectable } from '@angular/core';
import { map, switchMap } from 'rxjs/operators';
import { HttpClient, HttpErrorResponse } from '@angular/common/http';
import { environment } from '../environments/environment';
import { catchError } from 'rxjs/operators';
import { MessagesService } from './messages.service';
@Injectable({
providedIn: 'root'
})
export class FinanciacionService {
constructor(private http: HttpClient, private messageService: MessagesService) { }
removeFinanciacion(financiacion) {
return this.http.delete(`${environment.proyectoServiceHost}/api/financiacion/${financiacion.id}`)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
createFinanciacion(financiacion) {
return this.http.post(`${environment.proyectoServiceHost}/api/financiacion`, financiacion)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
modifyFinanciacion(financiacion) {
return this.http.put(`${environment.proyectoServiceHost}/api/financiacion/${financiacion.id}`, financiacion)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
}
<file_sep>/src/app/resultados/resultados.component.ts
import { Component, OnInit, Inject } from '@angular/core';
import { EscenariosService } from '../escenarios.service';
import { ActivatedRoute, Router } from '@angular/router';
import { UsuarioService } from '../usuario.service';
import { ProyectoService } from '../proyecto.service';
import { MatDialogRef, MAT_DIALOG_DATA, MatDialog } from '@angular/material';
@Component({
selector: 'app-resultados',
templateUrl: './resultados.component.html',
styleUrls: ['./resultados.component.css']
})
export class ResultadosComponent implements OnInit {
proyecto;
escenario;
decisiones;
escenarioCurso;
forecast;
seccion = "RESULTADOS_FORECAST";
constructor(private escenarioService: EscenariosService, private route: ActivatedRoute,
private usuarioService: UsuarioService, private proyectoService: ProyectoService, public dialog: MatDialog, private router: Router) { }
ngOnInit() {
var escenarioId = Number(this.route.snapshot.paramMap.get('escenarioId'));
this.getEscenario(escenarioId);
this.getProyecto(escenarioId, this.usuarioService.usuario.id);
this.getDetalleEscenarioUsuariosPorCurso(escenarioId, this.usuarioService.usuario.curso.id);
}
getDetalleEscenarioUsuariosPorCurso(escenarioId, cursoId) {
this.escenarioService.getDetalleEscenarioUsuariosPorCurso(escenarioId, cursoId).subscribe(escenarioCurso => {
this.escenarioCurso = escenarioCurso;
})
}
getProyecto(escenarioId, usuarioId) {
this.usuarioService.getProyecto(escenarioId, usuarioId).subscribe(estado => {
this.proyecto = estado.proyecto;
this.getDecisiones(this.proyecto.id);
this.getForecast(this.proyecto.id);
})
}
getDecisiones(proyectoId) {
this.proyectoService.getDecisiones(proyectoId)
.subscribe(decisiones => this.decisiones = decisiones);
}
getEscenario(escenarioId) {
this.escenarioService.getEscenario(escenarioId).subscribe(escenario => this.escenario = escenario);
}
getForecast(proyectoId) {
this.proyectoService.getForecast(proyectoId).subscribe(forecast => {this.forecast = forecast;
if(forecast.length <= 0) this.seccion = "RESULTADOS_REALES"});
}
entregarSimulacion() {
const dialogRef = this.dialog.open(EntregarConfirmationPopup, {
width: window.screen.width > 768 ? '50%' : '95%'
});
dialogRef.afterClosed().subscribe(respuesta => {
if (respuesta === "ENTREGAR") {
this.proyectoService.entregarProyecto(this.proyecto.id)
.subscribe( _ => this.router.navigate(['/simulacion-entregada']) );
}
});
}
}
@Component({
selector: 'entregar-confirmation-popup',
templateUrl: 'entregar-confirmation-popup.html',
})
export class EntregarConfirmationPopup {
constructor(
public dialogRef: MatDialogRef<EntregarConfirmationPopup>) { }
onEntregarClick(): void {
this.dialogRef.close('ENTREGAR');
}
onCancelClick(): void {
this.dialogRef.close();
}
}
<file_sep>/src/app/presupuesto-financiero/presupuesto-financiero.component.ts
import { Component, OnInit, Input } from '@angular/core';
import { ProyectoService } from '../proyecto.service';
import { ActivatedRoute } from '@angular/router';
import { UsuarioService } from '../usuario.service';
@Component({
selector: 'app-presupuesto-financiero',
templateUrl: './presupuesto-financiero.component.html',
styleUrls: ['./presupuesto-financiero.component.css']
})
export class PresupuestoFinancieroComponent implements OnInit {
@Input() proyecto: any;
@Input() forecast: boolean;
presupuestoFinanciero;
periodos;
escenario;
constructor(private proyectoService: ProyectoService) { }
ngOnInit() {
this.getPeriodos(this.proyecto.id);
this.getPresupuestoFinanciero(this.proyecto.id);
this.escenario = this.proyecto.escenario;
}
getPresupuestoFinanciero(proyectoId) {
this.proyectoService.getPresupuestoFinanciero(proyectoId,this.forecast).subscribe(pf => {
this.presupuestoFinanciero = pf;
})
}
getPeriodos(proyectoId) {
this.proyectoService.getPeriodoActual(proyectoId, this.forecast).subscribe(periodoActual => {
this.periodos = [...Array(periodoActual).keys(), periodoActual];
})
}
getCuentaPeriodo(cuentasPeriodo, periodo, defaultCero = true, signoInvertido = false) {
var periodo = cuentasPeriodo && cuentasPeriodo.find(cuentaPeriodo => cuentaPeriodo.periodo === periodo)
var montoCrudo = periodo ? periodo.monto : (defaultCero ? 0 : "");
montoCrudo = signoInvertido ? -montoCrudo : montoCrudo;
if (montoCrudo < 0) {
return "(" + Math.abs(montoCrudo).toLocaleString() + ")";
} else if (montoCrudo === 0) {
return montoCrudo;
} else {
return montoCrudo.toLocaleString();
}
}
getTotalAcumulado(cuentasPeriodo){
var totalAcumulado = cuentasPeriodo.reduce((total,cp) => total + cp.monto,0 );
if (totalAcumulado < 0) {
return "(" + Math.abs(totalAcumulado).toLocaleString() + ")";
} else if (totalAcumulado === 0) {
return totalAcumulado;
} else {
return totalAcumulado.toLocaleString();
}
}
}
<file_sep>/src/app/estado/estado.component.ts
import { Component, OnInit, Input } from '@angular/core';
import { ProyectoService } from "../proyecto.service";
import { Observable } from 'rxjs/Observable';
import * as Chart from 'chart.js';
import { UsuarioService } from '../usuario.service';
import { ActivatedRoute } from '@angular/router';
@Component({
selector: 'app-estado',
templateUrl: './estado.component.html',
styleUrls: ['./estado.component.css']
})
export class EstadoComponent implements OnInit {
@Input() proyecto: any;
@Input() forecast: boolean;
estadoActual: any;
ultimoPrecioProducto;
estados: any;
cajaChartProps;
ventasChartProps;
ventasVsStockChartProps;
escenario;
constructor(private proyectoService: ProyectoService,
private route: ActivatedRoute,
private usuarioService: UsuarioService) {
}
ngOnInit() {
this.getEstadoActual(this.proyecto.id);
this.getUltimoPrecioProducto(this.proyecto.id);
}
getUltimoPrecioProducto(proyectoId) {
if (this.forecast) {
this.proyectoService.getForecast(proyectoId).subscribe(forecasts => {
this.ultimoPrecioProducto = forecasts.pop().precio;
})
} else {
this.ultimoPrecioProducto = 0;
}
}
getPeriodoQuiebreCaja(estados) {
let estadoQuiebreCaja = estados.find(estado => estado.caja < 0);
return estados.indexOf(estadoQuiebreCaja);
}
getEstadoActual(proyectoId) {
this.estadoActual = this.proyectoService.getEstado(proyectoId, this.forecast).subscribe(estadoActual => {
this.estadoActual = estadoActual;
this.escenario = estadoActual.proyecto.escenario;
this.getEstados(proyectoId);
})
}
getEstados(proyectoId) {
this.proyectoService.getEstados(proyectoId, this.forecast).subscribe(estados => {
this.estados = estados;
this.setCajaChartProps(this.estados);
this.setVentasChartProps(this.estados);
this.proyectoService.getForecast(proyectoId).subscribe(forecasts => {
this.setStockVsVentasChartProps(this.estados, forecasts);
})
})
}
setCajaChartProps(estados) {
this.cajaChartProps = {
options: {
scaleShowVerticalLines: false,
responsive: true
},
labels: estados.map(estado => `${this.escenario.nombrePeriodos} ${estado.periodo}`),
type: 'line',
legend: false,
data: [
{ data: estados.map(estado => estado.caja), label: 'Caja', fill: false }
]
}
}
setVentasChartProps(estados) {
this.ventasChartProps = {
options: {
scaleShowVerticalLines: false,
responsive: true
},
labels: estados.filter((_, index) => index !== 0).map(estado => `${this.escenario.nombrePeriodos} ${estado.periodo}`),
type: 'line',
legend: true,
data: [
{
data: estados.filter((_, index) => index !== 0).map(estado => estado.ventas),
fill: false,
label: 'Ventas'
},
{
data: estados.filter((_, index) => index !== 0).map(estado => estado.demandaPotencial),
label: 'Demanda obtenida',
fill: false,
backgroundColor: 'rgb(75, 192, 192)',
borderColor: 'rgb(75, 192, 192)',
borderDash: [5, 5]
}
]
}
}
setStockVsVentasChartProps(estados, forecasts) {
var forecastForVentas = forecasts.filter((_, index) => index !== 0);
this.ventasVsStockChartProps = {
options: {
scaleShowVerticalLines: false,
responsive: true,
aspectRatio: 1.5
},
labels: estados.filter((_, index) => index !== 0).map(e => e.proyecto.escenario.nombrePeriodos + " " + e.periodo),
type: 'bar',
legend: true,
data: [
{
data: estados.filter((_, index) => index !== 0).map((e, index) => {
var precio = forecastForVentas[index].precio;
if (precio === 0) {
return 0;
} else {
return e.ventas / precio;
}
}),
type: 'line',
fill: false,
label: 'Cantidad Vendida'
},
{
data: estados.filter((_, index) => index !== 0).map(e => e.stock),
type: 'bar',
label: 'Stock',
fill: false
}
]
}
}
getEvenNumber = function (num) {
return Math.floor(Number(num) / 2) > 0 ? new Array(Math.floor(Number(num) / 2)) : new Array(0);
}
getOddNumber = function (num) {
return Number(num) % 2 != 0;
}
}
<file_sep>/src/app/estado-juegos/estado-juegos.component.ts
import { Component, OnInit } from '@angular/core';
import { EscenariosService } from '../escenarios.service';
import { CursosService } from '../cursos.service';
import { zip, of } from "rxjs";
import { switchMap, map, flatMap, combineAll } from 'rxjs/operators';
import { UsuarioService } from '../usuario.service';
@Component({
selector: 'app-estado-juegos',
templateUrl: './estado-juegos.component.html',
styleUrls: ['./estado-juegos.component.css']
})
export class EstadoJuegosComponent implements OnInit {
cursosTree;
escenarios: Array<any>;
usuario;
constructor(private escenariosService: EscenariosService,
private cursosService: CursosService,
private usuarioService: UsuarioService) { }
ngOnInit() {
this.getCursosPorEscenario();
this.usuario = this.usuarioService.usuario;
}
getCursosPorEscenario() {
const $cursos = this.cursosService.getCursos();
const $escenarios = this.escenariosService.getEscenarios();
const $escenariosConCursos = $escenarios.pipe(
switchMap(escenarios => {
const $escenarios = escenarios.map(escenario => {
return this.escenariosService.getCursosEscenario(escenario.id).pipe(
map(cursosPorEscenario => {
escenario.cursos = cursosPorEscenario
return escenario;
})
)
})
return zip(...$escenarios);
})
);
zip($cursos, $escenariosConCursos).subscribe(([cursos, escenariosConCursos]) => {
var cursosTree = cursos.map(curso => {
curso.escenarios = escenariosConCursos.filter((escenario: any) => escenario.cursos.find(c => c.nombre === curso.nombre));
curso.escenarios = JSON.parse(JSON.stringify(curso.escenarios));//Debo copiar la lista como un nuevo objeto para que existan varias referencias
return curso;
});
cursosTree.forEach(curso => {
curso.escenarios.forEach(escenario => {
this.escenariosService.getDetalleEscenarioUsuariosPorCurso(escenario.id, curso.id)
.subscribe(escenarioCurso => {
escenario.escenarioCurso = escenarioCurso
})
})
})
this.cursosTree = cursosTree;
})
}
}
<file_sep>/src/app/proveedores.service.ts
import { Injectable } from '@angular/core';
import { EscenariosService } from './escenarios.service';
import { map, switchMap } from 'rxjs/operators';
import { HttpClient, HttpErrorResponse } from '@angular/common/http';
import { environment } from '../environments/environment';
import { catchError } from 'rxjs/operators';
import { MessagesService } from './messages.service';
@Injectable({
providedIn: 'root'
})
export class ProveedoresService {
constructor(private http: HttpClient, private messageService: MessagesService, private escenarioService: EscenariosService) { }
_getProveedores(idEscenario) {
return this.escenarioService.getProveedores(idEscenario);
}
removeProveedor(proveedor) {
return this.http.delete(`${environment.proyectoServiceHost}/api/proveedores/${proveedor.id}`)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
createProveedor(proveedor) {
return this.http.post(`${environment.proyectoServiceHost}/api/proveedor`, proveedor)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
modifyProveedor(proveedor) {
return this.http.put(`${environment.proyectoServiceHost}/api/proveedores/${proveedor.id}`, proveedor)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
}
<file_sep>/src/app/estado-juegos/estado-juegos.component.spec.ts
import { async, ComponentFixture, TestBed } from '@angular/core/testing';
import { EstadoJuegosComponent } from './estado-juegos.component';
describe('EstadoJuegosComponent', () => {
let component: EstadoJuegosComponent;
let fixture: ComponentFixture<EstadoJuegosComponent>;
beforeEach(async(() => {
TestBed.configureTestingModule({
declarations: [ EstadoJuegosComponent ]
})
.compileComponents();
}));
beforeEach(() => {
fixture = TestBed.createComponent(EstadoJuegosComponent);
component = fixture.componentInstance;
fixture.detectChanges();
});
it('should create', () => {
expect(component).toBeTruthy();
});
});
<file_sep>/src/app/escenarios/escenarios.component.ts
import { Component, OnInit } from '@angular/core';
import { ProyectoService } from '../proyecto.service';
import { EscenariosService } from '../escenarios.service';
import { UsuarioService } from '../usuario.service';
import { ConfirmationDialogComponent } from '../confirmation-dialog/confirmation-dialog.component';
import { MatDialog } from '@angular/material';
import { switchMap, map } from 'rxjs/operators';
import { zip } from 'rxjs';
@Component({
selector: 'app-escenarios',
templateUrl: './escenarios.component.html',
styleUrls: ['./escenarios.component.css']
})
export class EscenariosComponent implements OnInit {
usuario;
escenarios: Array<any>;
constructor(private escenariosService: EscenariosService, private usuarioService: UsuarioService,
private dialog: MatDialog) { }
ngOnInit() {
this.usuario = this.usuarioService.usuario;
this.getEscenarios();
}
getEscenarios() {
this.escenariosService.getEscenarios().pipe(
switchMap(escenarios => {
const $escenarios = escenarios.map(e => {
return this.escenariosService.getProyectosByEscenario(e.id).pipe(
map(proyectos => {
e.hasProyectos = (proyectos.length > 0)
return e;
})
)
})
return zip(...$escenarios)
})
).subscribe(escenarios => this.escenarios = escenarios)
}
deleteEscenario(id) {
const dialogRef = this.dialog.open(ConfirmationDialogComponent, {
width: '500px',
data: {
message: "¿Seguro que desea eliminar el escenario?"
}
});
dialogRef.afterClosed().subscribe(response => {
if (response === "OK") {
this.escenariosService.deleteEscenario(id).subscribe(_ => this.getEscenarios())
}
});
}
duplicateEscenario(escenario) {
const dialogRef = this.dialog.open(ConfirmationDialogComponent, {
width: '500px',
data: {
message: "¿Seguro que desea clonar el escenario?"
}
});
dialogRef.afterClosed().subscribe(response => {
if (response === "OK") {
this.escenariosService.duplicateEscenario(escenario).subscribe(_ => this.getEscenarios())
}
});
}
}
<file_sep>/src/app/premios/premios.component.ts
import { Component, OnInit } from '@angular/core';
import { EscenariosService } from '../escenarios.service';
import { UsuarioService } from '../usuario.service';
import { switchMap, map, filter } from 'rxjs/operators';
import { zip } from 'rxjs';
@Component({
selector: 'app-premios',
templateUrl: './premios.component.html',
styleUrls: ['./premios.component.css']
})
export class PremiosComponent implements OnInit {
usuario;
escenarios;
constructor(private escenariosService: EscenariosService, private usuarioService: UsuarioService) { }
ngOnInit() {
this.usuario = this.usuarioService.usuario;
this.getEscenarios(this.usuario.id, this.usuario.curso.id);
}
getEscenarios(idUsuario, cursoId) {
const $escenarios = this.escenariosService.getEscenariosParaUsuario(idUsuario);
const $escenariosConProyectos = $escenarios.pipe(
switchMap(escenarios => {
const $escenarios = escenarios.map(escenario => {
var $proyecto = this.usuarioService.getProyecto(escenario.id, idUsuario);
var $escenarioCurso = this.escenariosService.getDetalleEscenarioUsuariosPorCurso(escenario.id, cursoId);
return zip($proyecto, $escenarioCurso).pipe(
map(([proyecto, escenarioCurso]) => {
escenario.proyecto = proyecto;
escenario.escenarioCurso = escenarioCurso;
escenario.podio = this._jugadoresToPodio(escenarioCurso.jugadores);
return escenario;
})
);
})
return zip(...$escenarios);
}));
$escenariosConProyectos.subscribe(escenarios => this.escenarios = escenarios);
}
_jugadoresToPodio(jugadores) {
return {
primerPuesto: jugadores[0],
segundoPuesto: jugadores[1],
tercerPuesto: jugadores[2]
}
}
}
<file_sep>/src/app/decision-detalle/opciones/opcion-dialog.component.ts
import { Component, OnInit, Inject } from '@angular/core';
import { MatDialogRef, MAT_DIALOG_DATA } from '@angular/material';
import { FormControl, Validators, FormGroup } from '@angular/forms';
import { MessagesService } from '../../messages.service';
@Component({
selector: 'opcion-dialog',
templateUrl: './opcion-dialog.component.html',
styleUrls: ['./opcion-dialog.component.css']
})
export class OpcionDialogComponent implements OnInit {
opcion;
descripcion = new FormControl('', [Validators.required])
variacionCostoFijo = new FormControl('', [Validators.required])
variacionCostoVariable = new FormControl('', [Validators.required])
variacionProduccion = new FormControl('', [Validators.required])
variacionCalidad = new FormControl('', [Validators.required])
variacionPublicidad = new FormControl('', [Validators.required])
variacionCantidadVendedores = new FormControl('', [Validators.required])
opcionForm: FormGroup = new FormGroup({
descripcion: this.descripcion,
variacionCostoFijo: this.variacionCostoFijo,
variacionCostoVariable: this.variacionCostoVariable,
variacionProduccion: this.variacionProduccion,
variacionCalidad: this.variacionCalidad,
variacionPublicidad: this.variacionPublicidad,
variacionCantidadVendedores: this.variacionCantidadVendedores
});
constructor( public dialogRef: MatDialogRef<OpcionDialogComponent>,
private messageService: MessagesService,
@Inject(MAT_DIALOG_DATA) public data) { }
ngOnInit() {
this.opcion = JSON.parse(JSON.stringify(this.data));
}
cancel(){
this.dialogRef.close();
}
save(){
if(this.opcionForm.valid){
this.dialogRef.close(this.opcion);
}else {
this.messageService.openSnackBarDatosIngresados()
}
}
}
<file_sep>/src/app/estado-juego-detail/estado-juego-detail.component.spec.ts
import { async, ComponentFixture, TestBed } from '@angular/core/testing';
import { EstadoJuegoDetailComponent } from './estado-juego-detail.component';
describe('EstadoJuegoDetailComponent', () => {
let component: EstadoJuegoDetailComponent;
let fixture: ComponentFixture<EstadoJuegoDetailComponent>;
beforeEach(async(() => {
TestBed.configureTestingModule({
declarations: [ EstadoJuegoDetailComponent ]
})
.compileComponents();
}));
beforeEach(() => {
fixture = TestBed.createComponent(EstadoJuegoDetailComponent);
component = fixture.componentInstance;
fixture.detectChanges();
});
it('should create', () => {
expect(component).toBeTruthy();
});
});
<file_sep>/src/app/login/login.component.ts
import { Component, OnInit, HostBinding } from '@angular/core';
import { AngularFireAuth } from '@angular/fire/auth';
import * as firebase from 'firebase/app';
import { Router } from '@angular/router';
import { DomSanitizer } from '@angular/platform-browser';
import { MatIconRegistry, MatSnackBar } from '@angular/material';
import { MatDialog, DialogPosition } from '@angular/material';
import { RegisterComponent } from './components/register.component';
import { FormControl, Validators, FormGroup } from '@angular/forms';
@Component({
selector: 'app-login',
templateUrl: './login.component.html',
styleUrls: ['./login.component.css']
})
export class LoginComponent implements OnInit {
hide = true;
rememberMeChecked = true;
persistance = firebase.auth.Auth.Persistence.LOCAL;
loading = false;
email = new FormControl('', [Validators.required, Validators.email])
password = new FormControl('', [Validators.required])
registerForm: FormGroup = new FormGroup({
email: this.email,
password: this.password,
});
constructor(private afAuth: AngularFireAuth,
private router: Router,
iconRegistry: MatIconRegistry,
sanitizer: DomSanitizer,
private dialog: MatDialog,
public snackBar: MatSnackBar) {
this.loading = true;
this.afAuth.authState.subscribe(user => {
this.loading = false;
if (user) {
this.router.navigateByUrl("/home");
}
});
iconRegistry.addSvgIcon('facebook_icon', sanitizer.bypassSecurityTrustResourceUrl('assets/icons/facebook-logo.svg'));
iconRegistry.addSvgIcon('google_icon', sanitizer.bypassSecurityTrustResourceUrl('assets/icons/google-plus.svg'));
}
ngOnInit() {
}
withGoogle() {
this.afAuth.auth.setPersistence(this.persistance)
.then(_ => this.afAuth.auth.signInWithRedirect(new firebase.auth.GoogleAuthProvider())
.catch(e => this.openSnackBar(e.message)))
.catch(e => this.openSnackBar(e.message));
}
withFacebook() {
this.afAuth.auth.setPersistence(this.persistance)
.then(_ => this.afAuth.auth.signInWithRedirect(new firebase.auth.FacebookAuthProvider())
.catch(e => this.openSnackBar(e.message)))
.catch(e => this.openSnackBar(e.message));
}
withEmail() {
if (this.registerForm.valid) {
this.afAuth.auth.setPersistence(this.persistance)
.then(_ => this.afAuth.auth.signInWithEmailAndPassword(this.email.value, this.password.value)
.catch(e => this.openSnackBar(e.message)))
.catch(e => this.openSnackBar(e.message));
}
}
rememberMe() {
if (this.rememberMeChecked) {
this.persistance = firebase.auth.Auth.Persistence.LOCAL;
} else {
this.persistance = firebase.auth.Auth.Persistence.SESSION;
}
}
openRegisterDialog() {
const dialogRef = this.dialog.open(RegisterComponent, {
height: '350px'
});
dialogRef.afterClosed().subscribe(result => {
console.log(`Dialog result: ${result}`);
});
}
openSnackBar(message: string, action: string = "OK") {
this.snackBar.open(message, action, {
duration: 2000,
//extraClasses: ['error-snack-bar']
});
}
}
<file_sep>/src/app/financiaciones/financiacion-dialog/financiacion-dialog.component.ts
import { Component, OnInit, Inject } from '@angular/core';
import { MatDialogRef, MAT_DIALOG_DATA } from '@angular/material';
import { FormControl, Validators, FormGroup } from '@angular/forms';
import { MessagesService } from '../../messages.service';
import { Router } from '@angular/router';
import { FinanciacionService } from '../../financiacion.service';
@Component({
selector: 'financiacion-dialog',
templateUrl: './financiacion-dialog.component.html',
styleUrls: ['./financiacion-dialog.component.css']
})
export class FinanciacionDialogComponent implements OnInit {
financiacion;
descripcion = new FormControl('', [Validators.required])
tea = new FormControl('', [Validators.required, Validators.min(1), Validators.max(100)])
cantidadCuotas = new FormControl('', [Validators.required])
financiacionForm: FormGroup = new FormGroup({
descripcion: this.descripcion,
cantidadCuotas: this.cantidadCuotas,
tea: this.tea
});
constructor( public dialogRef: MatDialogRef<FinanciacionDialogComponent>,
@Inject(MAT_DIALOG_DATA) public data, private financiacionService: FinanciacionService,
private messageService: MessagesService, private router: Router) { }
ngOnInit() {
this.financiacion = JSON.parse(JSON.stringify(this.data));
}
cancel(){
this.dialogRef.close();
}
save() {
if (this.financiacionForm.valid) {
this.financiacionService[this.financiacion.id ? 'modifyFinanciacion' : 'createFinanciacion'](this.financiacion)
.subscribe(_ => {
this.messageService.openSnackBar("Financiacion modificada");
this.dialogRef.close();
})
}
}
}
<file_sep>/src/app/valores-dominio.ts
export const descripcionesTipoCuentas = {
"ECONOMICO": "Economico",
"FINANCIERO": "Financiero"
};
export const descripcionesTipoFlujoFondos = {
"INGRESOS_AFECTOS_A_IMPUESTOS": "Ingresos afectos a impuestos",
"INGRESOS_NO_AFECTOS_A_IMPUESTOS": "Ingresos no afectos a impuestos",
"GASTOS_NO_DESEMBOLSABLES": "Gastos no desembolsables",
"EGRESOS_AFECTOS_A_IMPUESTOS": "Egresos afectos a impuestos",
"EGRESOS_NO_AFECTOS_A_IMPUESTOS": "Egresos no afectos a impuestos",
"UTILIDAD_ANTES_DE_IMPUESTOS": "Utilidad antes de impuestos",
"IMPUESTOS": "Impuestos",
"INVERSIONES": "Inversiones",
"UTILIDAD_DESPUES_DE_IMPUESTOS": "Utilidad despues de impuestos",
"AJUSTE_DE_GASTOS_NO_DESEMBOLSABLES": "Ajuste de gastos no desembolsables",
"FLUJO_DE_FONDOS": "Flujo de fondos"
};
<file_sep>/src/app/balance-final/balance-final.component.ts
import { Component, OnInit, Input } from '@angular/core';
import { ProyectoService } from '../proyecto.service';
@Component({
selector: 'app-balance-final',
templateUrl: './balance-final.component.html',
styleUrls: ['./balance-final.component.css']
})
export class BalanceFinalComponent implements OnInit {
@Input() proyecto: any;
@Input() forecast: boolean;
balanceFinal;
totalActivo = 0;
totalPasivoPatrimonioNeto = 0;
constructor(private proyectoService: ProyectoService) { }
ngOnInit() {
this.getBalanceFinal(this.proyecto.id);
}
getBalanceFinal(idProyecto){
this.proyectoService.getBalanceFinal(idProyecto,this.forecast).subscribe(balanceFinal => {this.balanceFinal = balanceFinal; this.getTotalActivo(); this.getTotalPasivoPatrimonioNeto()});
}
getTotalActivo() {
this.totalActivo = this.balanceFinal.activo.caja + this.balanceFinal.activo.cuentasPorCobrar + this.balanceFinal.activo.inventario + this.balanceFinal.activo.maquinaria + this.balanceFinal.activo.amortizacionAcumulada + this.balanceFinal.activo.otros;
}
getTotalPasivoPatrimonioNeto() {
this.totalPasivoPatrimonioNeto = this.balanceFinal.pasivo.proveedores + this.balanceFinal.pasivo.deudasBancarias + this.balanceFinal.pasivo.otros + this.balanceFinal.patrimonioNeto.capitalSocial + this.balanceFinal.patrimonioNeto.resultadoDelEjercicio;
}
}
<file_sep>/src/app/decisiones/decisiones.component.ts
import { Component, OnInit, Input } from '@angular/core';
import { DecisionesService } from '../decisiones.service';
import { EscenariosService } from '../escenarios.service';
@Component({
selector: 'app-decisiones',
templateUrl: './decisiones.component.html',
styleUrls: ['./decisiones.component.css']
})
export class DecisionesComponent implements OnInit {
@Input() escenario: any;
@Input() hasProyectos: boolean;
decisiones: Array<any>;
constructor(private decisionesService: DecisionesService, private escenariosService: EscenariosService) { }
ngOnInit() {
this.getDecisiones();
}
getDecisiones() {
this.escenariosService.getDecisiones(this.escenario.id).subscribe( decisiones => {
this.decisiones = decisiones;
});
}
removeDecision(id){
this.decisionesService.removeDecision(id).subscribe(_ => this.getDecisiones());
}
}
<file_sep>/src/app/rankings/rankings.component.ts
import { Component, OnInit, Input } from '@angular/core';
import { ActivatedRoute } from '@angular/router';
import { CursosService } from '../cursos.service';
import { EscenariosService } from '../escenarios.service';
import { MessagesService } from '../messages.service';
import { UsuarioService } from '../usuario.service';
@Component({
selector: 'app-rankings',
templateUrl: './rankings.component.html',
styleUrls: ['./rankings.component.css']
})
export class RankingsComponent implements OnInit {
@Input() escenario: any;
@Input() curso: any;
ventasChartProps;
cajaChartProps;
rentaChartProps;
escenarioCurso;
puntajes;
constructor(private route: ActivatedRoute, private cursosService: CursosService,
private escenariosService: EscenariosService,
private usuarioService: UsuarioService) { }
ngOnInit() {
this.getCurso(this.usuarioService.usuario.cursoId);
this.getDetalleEscenarioUsuariosPorCurso(this.escenario.id, this.curso.id);
this.getPuntajes(this.escenario.id)
}
getCurso(cursoId) {
this.cursosService.getCurso(cursoId).subscribe(curso => this.curso = curso)
}
getDetalleEscenarioUsuariosPorCurso(escenarioId, cursoId) {
this.escenariosService.getDetalleEscenarioUsuariosPorCurso(escenarioId, cursoId).subscribe(escenarioCurso => {
this.escenarioCurso = escenarioCurso;
this.setVentasChartProps(escenarioCurso.jugadores);
this.setCajaChartProps(escenarioCurso.jugadores);
this.setRentaChartProps(escenarioCurso.jugadores);
})
}
getPuntajes(escenarioId) {
this.escenariosService.getPuntajes(escenarioId).subscribe(puntajes => {
if (puntajes) {
this.puntajes = puntajes;
}
})
}
setVentasChartProps(jugadores) {
var jugadoresPorVentas = jugadores.concat([]);
jugadoresPorVentas.sort((j1, j2) => j1.ventas < j2.ventas);
this.ventasChartProps = {
options: {
scaleShowVerticalLines: false,
responsive: true,
aspectRatio: 3
},
labels: jugadoresPorVentas.map(j => j.usuarioNombre),
type: 'horizontalBar',
legend: false,
data: [
{
data: jugadoresPorVentas.map(j => j.ventasTotales),
label: 'Ventas'
}
]
}
}
setCajaChartProps(jugadores) {
var jugadoresPorCaja = jugadores.concat([]);
jugadoresPorCaja.sort((j1, j2) => j1.cajaFinal < j2.cajaFinal);
this.cajaChartProps = {
options: {
scaleShowVerticalLines: false,
responsive: true,
aspectRatio: 3
},
labels: jugadoresPorCaja.map(j => j.usuarioNombre),
type: 'horizontalBar',
legend: false,
data: [
{
data: jugadoresPorCaja.map(j => j.cajaFinal),
label: 'Caja Final'
}
]
}
}
setRentaChartProps(jugadores) {
var jugadoresPorRenta = jugadores.concat([]);
jugadoresPorRenta.sort((j1, j2) => j1.renta < j2.renta);
this.rentaChartProps = {
options: {
scaleShowVerticalLines: false,
responsive: true,
aspectRatio: 3
},
labels: jugadoresPorRenta.map(j => j.usuarioNombre),
type: 'horizontalBar',
legend: false,
data: [
{
data: jugadoresPorRenta.map(j => j.renta),
label: 'Renta'
}
]
}
}
getMedalUrlByIndex(index) {
switch (index) {
case 0: return "../../assets/img/gold-medal.png";
case 1: return "../../assets/img/silver-medal.png";
case 2: return "../../assets/img/bronze-medal.png";
default: return "../../assets/img/medal.png";
}
}
}
<file_sep>/src/app/premios-detail/premios-detail.component.spec.ts
import { async, ComponentFixture, TestBed } from '@angular/core/testing';
import { PremiosDetailComponent } from './premios-detail.component';
describe('PremiosDetailComponent', () => {
let component: PremiosDetailComponent;
let fixture: ComponentFixture<PremiosDetailComponent>;
beforeEach(async(() => {
TestBed.configureTestingModule({
declarations: [ PremiosDetailComponent ]
})
.compileComponents();
}));
beforeEach(() => {
fixture = TestBed.createComponent(PremiosDetailComponent);
component = fixture.componentInstance;
fixture.detectChanges();
});
it('should create', () => {
expect(component).toBeTruthy();
});
});
<file_sep>/src/environments/environment.prod.ts
export const environment = {
production: true,
firebase: {
apiKey: "<KEY>",
authDomain: "augure-8db58.firebaseapp.com",
databaseURL: "https://augure-8db58.firebaseio.com",
projectId: "augure-8db58",
storageBucket: "",
messagingSenderId: "30865807746"
},
proyectoServiceHost: ""
};
<file_sep>/src/app/decision-detalle/consecuencias/consecuencia-dialog.component.ts
import { Component, OnInit, Inject } from '@angular/core';
import { MatDialogRef, MAT_DIALOG_DATA } from '@angular/material';
import { FormControl, Validators, FormGroup } from '@angular/forms';
import { ProyectoService } from '../../proyecto.service';
import { MessagesService } from '../../messages.service';
@Component({
selector: 'consecuencia-dialog',
templateUrl: './consecuencia-dialog.component.html',
styleUrls: ['./consecuencia-dialog.component.css']
})
export class ConsecuenciaDialogComponent implements OnInit {
tipoCuentas;
tipoFlujoFondos;
consecuencia;
descripcion = new FormControl('', [Validators.required])
monto = new FormControl('', [Validators.required])
periodoInicio = new FormControl('', [Validators.required])
cantidadPeriodos = new FormControl('', [Validators.required])
tipoCuenta = new FormControl('', [Validators.required])
tipoFlujoFondo = new FormControl('', [Validators.required])
tipoBalance = new FormControl(null);
consecuenciaForm: FormGroup = new FormGroup({
descripcion: this.descripcion,
monto: this.monto,
periodoInicio: this.periodoInicio,
cantidadPeriodos: this.cantidadPeriodos,
tipoCuenta: this.tipoCuenta,
tipoFlujoFondo: this.tipoFlujoFondo,
tipoBalance: this.tipoBalance
});
constructor(public dialogRef: MatDialogRef<ConsecuenciaDialogComponent>,
private proyectoService: ProyectoService,
private messageService: MessagesService,
@Inject(MAT_DIALOG_DATA) public data) { }
ngOnInit() {
this.getTipoCuentas();
this.getTipoFlujoFondos();
this.consecuencia = JSON.parse(JSON.stringify(this.data));
}
getTipoCuentas(){
this.proyectoService.getTipoCuentas().subscribe(tipoCuentas => this.tipoCuentas = tipoCuentas)
}
getTipoFlujoFondos(){
this.proyectoService.getTipoFlujoFondos().subscribe(tipoFlujoFondos => this.tipoFlujoFondos = tipoFlujoFondos)
}
cancel(){
this.dialogRef.close();
}
save(){
if(this.consecuenciaForm.valid){
this.dialogRef.close(this.consecuencia);
}else {
this.messageService.openSnackBarDatosIngresados()
}
}
}
<file_sep>/src/app/cursos/cursos.component.ts
import { Component, OnInit } from '@angular/core';
import { CursosService } from '../cursos.service';
import { CursoDialogComponent } from './curso-dialog/curso-dialog.component';
import { MatDialog } from '@angular/material';
import { UsuarioService } from '../usuario.service';
import { ConfirmationDialogComponent } from '../confirmation-dialog/confirmation-dialog.component';
@Component({
selector: 'app-cursos',
templateUrl: './cursos.component.html',
styleUrls: ['./cursos.component.css']
})
export class CursosComponent implements OnInit {
cursos;
usuario;
constructor(private cursosService: CursosService, private dialog: MatDialog, private usuarioService: UsuarioService) { }
ngOnInit() {
this.getCursos();
this.usuario = this.usuarioService.usuario;
}
getCursos() {
this.cursosService.getCursos().subscribe(cursos => this.cursos = cursos.map(
c => {
const curso={
id: c.id,
nombre: c.nombre,
clave: atob(c.clave)
};
return curso;
}
));
}
decodeBase64() {
}
editCurso(curso) {
const dialogRef = this.dialog.open(CursoDialogComponent, {
width: '400px',
data: curso
});
dialogRef.afterClosed().subscribe(_ => this.getCursos());
}
removeCurso(curso) {
const dialogRef = this.dialog.open(ConfirmationDialogComponent, {
width: '500px',
data: {
message: "Seguro que desea eliminar el curso?"
}
});
dialogRef.afterClosed().subscribe(response => {
if (response === "OK") {
this.cursosService.removeCurso(curso).subscribe(_ => this.getCursos())
}
});
}
createCurso() {
const dialogRef = this.dialog.open(CursoDialogComponent, {
width: '400px',
data: {
nombre: "",
clave: ""
}
});
dialogRef.afterClosed().subscribe(_ => this.getCursos());
}
}
<file_sep>/src/app/decisiones.service.ts
import { Injectable } from '@angular/core';
import { HttpClient, HttpErrorResponse } from '@angular/common/http';
import { environment } from '../environments/environment';
import { catchError } from 'rxjs/operators';
import { MessagesService } from './messages.service';
@Injectable({
providedIn: 'root'
})
export class DecisionesService {
constructor(private http: HttpClient, private messageService: MessagesService) { }
createDecision(decision) {
return this.http.post(`${environment.proyectoServiceHost}/api/decisiones`, decision)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
modifyDecision(decision) {
return this.http.put(`${environment.proyectoServiceHost}/api/decisiones/${decision.id}`, decision)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
removeDecision(idDecision) {
return this.http.delete(`${environment.proyectoServiceHost}/api/decisiones/${idDecision}`)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
}
<file_sep>/src/app/confirmation-dialog/confirmation-dialog.component.ts
import { Component, OnInit, Inject } from '@angular/core';
import { MatDialogRef, MAT_DIALOG_DATA } from '@angular/material';
import { MessagesService } from '../messages.service';
@Component({
selector: 'app-confirmation-dialog',
templateUrl: './confirmation-dialog.component.html',
styleUrls: ['./confirmation-dialog.component.css']
})
export class ConfirmationDialogComponent implements OnInit {
message;
constructor(public dialogRef: MatDialogRef<ConfirmationDialogComponent>,
private messageService: MessagesService,
@Inject(MAT_DIALOG_DATA) public data) { }
ngOnInit() {
const data = JSON.parse(JSON.stringify(this.data));
this.message = data.message;
}
cancel() {
this.dialogRef.close();
}
accept() {
this.dialogRef.close("OK");
}
}
<file_sep>/src/app/estado-juego-detail/estado-juego-detail.component.ts
import { Component, OnInit } from '@angular/core';
import { ActivatedRoute } from '@angular/router';
import { CursosService } from '../cursos.service';
import { EscenariosService } from '../escenarios.service';
import { Message, MessageSpan } from '@angular/compiler/src/i18n/i18n_ast';
import { MessagesService } from '../messages.service';
import { FormControl, FormGroup, Validators } from '@angular/forms';
import { MatDialog } from '@angular/material';
import { ConfirmationDialogComponent } from '../confirmation-dialog/confirmation-dialog.component';
@Component({
selector: 'app-estado-juego-detail',
templateUrl: './estado-juego-detail.component.html',
styleUrls: ['./estado-juego-detail.component.css']
})
export class EstadoJuegoDetailComponent implements OnInit {
escenario;
curso;
ventasChartProps;
escenarioCurso;
puntajes
configuracionMercado;
constructor(private route: ActivatedRoute, private cursosService: CursosService,
private escenariosService: EscenariosService,
private dialog: MatDialog,
private messageService: MessagesService) { }
ngOnInit() {
this._loadData()
}
_loadData() {
const escenarioId = Number(this.route.snapshot.paramMap.get('escenarioId'));
const cursoId = Number(this.route.snapshot.paramMap.get('cursoId'));
this.getCurso(cursoId)
this.getEscenario(escenarioId);
this.getDetalleEscenarioUsuariosPorCurso(escenarioId, cursoId);
this.getPuntajes(escenarioId)
}
getPuntajes(escenarioId) {
this.escenariosService.getPuntajes(escenarioId).subscribe(puntajes => {
if (puntajes) {
this.puntajes = puntajes;
}
})
}
getConfiguracionMercado(escenario) {
this.escenariosService.getConfiguracionMercado(escenario.id).subscribe(configuracionMercado => {
if (configuracionMercado.restriccionPrecio) {
this.configuracionMercado = configuracionMercado;
} else {
this.setConfiguracionMercado(escenario)
}
})
}
setConfiguracionMercado(escenario) {
const arrayPeriodos = [...Array(escenario.maximosPeriodos).keys()],
array3 = [...Array(3).keys()],
array4 = [...Array(4).keys()];
this.configuracionMercado = {
empresasCompetidoras: array3.map((_, index) => {
return {
escenarioId: escenario.id,
nombre: null,
bajo: 0,
medio: 0,
alto: 0
}
}),
mercadosPeriodo: arrayPeriodos.map((_, index) => {
return {
escenarioId: escenario.id,
periodo: index + 1,
bajo: 0,
medio: 0,
alto: 0
}
}),
ponderacionesMercado: {
precioDesde: array3.map((_, index) => {
return {
escenarioId: escenario.id,
concepto: "PRECIO_DESDE",
valor: 0,
bajo: 0,
medio: 0,
alto: 0
}
}),
vendedoresDesde: array3.map((_, index) => {
return {
escenarioId: escenario.id,
concepto: "VENDEDORES_DESDE",
valor: 0,
bajo: 0,
medio: 0,
alto: 0
}
}),
publicidadDesde: array3.map((_, index) => {
return {
escenarioId: escenario.id,
concepto: "PUBLICIDAD_DESDE",
valor: 0,
bajo: 0,
medio: 0,
alto: 0
}
}),
modalidadCobro: array4.map((_, index) => {
return {
escenarioId: escenario.id,
concepto: "MODALIDAD_DE_COBRO",
valor: index,
bajo: 0,
medio: 0,
alto: 0
}
}),
calidadDesde: array3.map((_, index) => {
return {
escenarioId: escenario.id,
concepto: "CALIDAD_DESDE",
valor: 0,
bajo: 0,
medio: 0,
alto: 0
}
})
},
restriccionPrecio: {
escenarioId: escenario.id,
precioMin: 0,
precioMax: 0
}
}
}
getModalidadCobroDesc(modCobroIndex) {
return ["Contado", "a 30 dias", "a 60 dias", "a 90 dias"][modCobroIndex]
}
getCurso(cursoId) {
this.cursosService.getCurso(cursoId).subscribe(curso => this.curso = curso)
}
getEscenario(escenarioId) {
this.escenariosService.getEscenario(escenarioId).subscribe(escenario => {
this.escenario = escenario
this.getConfiguracionMercado(escenario)
})
}
getDetalleEscenarioUsuariosPorCurso(escenarioId, cursoId) {
this.escenariosService.getDetalleEscenarioUsuariosPorCurso(escenarioId, cursoId).subscribe(escenarioCurso => this.escenarioCurso = escenarioCurso)
}
cerrarEscenario() {
const dialogRef = this.dialog.open(ConfirmationDialogComponent, {
width: '500px',
data: {
message: "¿Seguro que desea cerrar el escenario?"
}
});
dialogRef.afterClosed().subscribe(response => {
if (response === "OK") {
this.escenariosService.getConfiguracionMercado(this.escenario.id).subscribe(configuracionMercado => {
if (configuracionMercado.restriccionPrecio) {
this.escenariosService.simularMercado(this.escenario.id, this.curso.id).subscribe(_ => {
this.messageService.openSnackBar("Simulación de Mercado ejecutada correctamente")
this._loadData()
})
} else {
this.messageService.openSnackBar("Antes de cerrar el escenario, debe guardar las configuraciones de mercado")
}
})
}
});
}
}
<file_sep>/src/app/simulaciones/simulaciones.component.ts
import { Component, OnInit } from '@angular/core';
import { EscenariosService } from '../escenarios.service';
import { UsuarioService } from '../usuario.service';
import { zip, of } from "rxjs";
import { switchMap, map } from 'rxjs/operators';
import { Router } from '@angular/router';
@Component({
selector: 'app-simulaciones',
templateUrl: './simulaciones.component.html',
styleUrls: ['./simulaciones.component.css']
})
export class SimulacionesComponent implements OnInit {
usuario;
escenarios;
constructor(private escenariosService: EscenariosService, private usuarioService: UsuarioService,private router: Router) { }
ngOnInit() {
this.usuario = this.usuarioService.usuario;
this.getEscenarios(this.usuario.id);
if(this.usuario.rol === "ADMIN"){
this.router.navigateByUrl("/estado-juegos");
}
}
getEscenarios(idUsuario) {
const $escenarios = this.escenariosService.getEscenariosParaUsuario(idUsuario);
const $escenariosConProyectos = $escenarios.pipe(
switchMap(escenarios => {
const $escenarios = escenarios.map(escenario => {
var $proyecto = this.usuarioService.getProyecto(escenario.id, idUsuario);
var $escenarioCurso = this.escenariosService.getDetalleEscenarioUsuariosPorCurso(escenario.id, this.usuario.curso.id);
return zip($proyecto, $escenarioCurso).pipe(
map(([proyecto, escenarioCurso]) => {
escenario.proyecto = proyecto;
escenario.escenarioCurso = escenarioCurso;
return escenario;
})
);
})
return zip(...$escenarios);
}));
$escenariosConProyectos.subscribe(escenarios => this.escenarios = escenarios);
}
}
<file_sep>/src/app/app.module.ts
import '../polyfills';
import { platformBrowserDynamic } from '@angular/platform-browser-dynamic';
import { BrowserModule } from '@angular/platform-browser';
import { BrowserAnimationsModule } from '@angular/platform-browser/animations';
import { NgModule } from '@angular/core';
import { FormsModule, ReactiveFormsModule } from '@angular/forms';
import { AngularFireModule } from '@angular/fire';
import { AngularFireAuthModule } from '@angular/fire/auth';
import { environment } from '../environments/environment';
import { HttpClientModule } from '@angular/common/http';
import { CdkTableModule } from '@angular/cdk/table';
import { AppComponent } from './app.component';
import { AppRoutingModule } from './/app-routing.module';
import { EstadoComponent } from './estado/estado.component';
import { PremiosComponent } from './premios/premios.component';
import { HomeComponent } from './home/home.component';
import { LoginComponent } from './login/login.component';
import { RegisterComponent } from './login/components/register.component';
import { DecisionesComponent } from './decisiones/decisiones.component';
import { DecisionDetalleComponent } from './decision-detalle/decision-detalle.component';
import { FlujoFondosComponent } from './flujo-fondos/flujo-fondos.component';
import { ProyectoService } from './proyecto.service';
import { UsuarioService } from './usuario.service';
import { TomaDecisionesComponent } from './toma-decisiones/toma-decisiones.component';
import { ResultadosComponent, EntregarConfirmationPopup } from './resultados/resultados.component';
import { ResultadosAdminComponent } from './resultados-admin/resultados-admin.component';
import { EscenariosComponent } from './escenarios/escenarios.component';
import { EscenarioDetalleComponent } from './escenario-detalle/escenario-detalle.component';
import { ChartsModule } from 'ng2-charts';
import { EscenariosService } from './escenarios.service';
import { DecisionesService } from './decisiones.service';
import { OpcionDialogComponent } from './decision-detalle/opciones/opcion-dialog.component';
import { ConsecuenciaDialogComponent } from './decision-detalle/consecuencias/consecuencia-dialog.component';
import { MessagesService } from './messages.service';
import { SimulacionesComponent } from './simulaciones/simulaciones.component';
import { PresupuestoFinancieroComponent } from './presupuesto-financiero/presupuesto-financiero.component';
import { PresupuestoEconomicoComponent } from './presupuesto-economico/presupuesto-economico.component';
import { BalanceFinalComponent } from './balance-final/balance-final.component';
import { ProveedoresComponent } from './proveedores/proveedores.component';
import { ProveedorDetailComponent } from './proveedor-detail/proveedor-detail.component';
import { ProveedoresService } from './proveedores.service';
import { EstadoJuegosComponent } from './estado-juegos/estado-juegos.component';
import { FinanciacionesComponent } from './financiaciones/financiaciones.component';
import { FinanciacionService } from './financiacion.service';
import { FinanciacionDialogComponent } from './financiaciones/financiacion-dialog/financiacion-dialog.component';
import { CursosComponent } from './cursos/cursos.component';
import { CursoDialogComponent } from './cursos/curso-dialog/curso-dialog.component';
import {
MatAutocompleteModule,
MatButtonModule,
MatButtonToggleModule,
MatCardModule,
MatCheckboxModule,
MatChipsModule,
MatDatepickerModule,
MatDialogModule,
MatExpansionModule,
MatGridListModule,
MatIconModule,
MatInputModule,
MatListModule,
MatMenuModule,
MatNativeDateModule,
MatPaginatorModule,
MatProgressBarModule,
MatProgressSpinnerModule,
MatRadioModule,
MatRippleModule,
MatSelectModule,
MatSidenavModule,
MatSliderModule,
MatSlideToggleModule,
MatSnackBarModule,
MatSortModule,
MatTableModule,
MatTabsModule,
MatToolbarModule,
MatTooltipModule,
MatStepperModule,
MatTabGroup
} from '@angular/material';
import { MatriculacionDialogComponent } from './matriculacion-dialog/matriculacion-dialog.component';
import { EstadoJuegoDetailComponent } from './estado-juego-detail/estado-juego-detail.component';
import { SimulacionEntregadaComponent } from './simulacion-entregada/simulacion-entregada.component';
import { PremiosDetailComponent } from './premios-detail/premios-detail.component';
import { RankingsComponent } from './rankings/rankings.component';
import { ConfirmationDialogComponent } from './confirmation-dialog/confirmation-dialog.component';
@NgModule({
exports: [
CdkTableModule,
MatAutocompleteModule,
MatButtonModule,
MatButtonToggleModule,
MatCardModule,
MatCheckboxModule,
MatChipsModule,
MatStepperModule,
MatDatepickerModule,
MatDialogModule,
MatExpansionModule,
MatGridListModule,
MatIconModule,
MatInputModule,
MatListModule,
MatMenuModule,
MatNativeDateModule,
MatPaginatorModule,
MatProgressBarModule,
MatProgressSpinnerModule,
MatRadioModule,
MatRippleModule,
MatSelectModule,
MatSidenavModule,
MatSliderModule,
MatSlideToggleModule,
MatSnackBarModule,
MatSortModule,
MatTableModule,
MatTabsModule,
MatToolbarModule,
MatTooltipModule
],
imports: [AppRoutingModule]
})
export class MaterialModule { }
@NgModule({
declarations: [
AppComponent,
EstadoComponent,
PremiosComponent,
HomeComponent,
LoginComponent,
RegisterComponent,
DecisionesComponent,
DecisionDetalleComponent,
SimulacionEntregadaComponent,
FlujoFondosComponent,
TomaDecisionesComponent,
ResultadosComponent,
ResultadosAdminComponent,
EscenariosComponent,
EscenarioDetalleComponent,
OpcionDialogComponent,
ConsecuenciaDialogComponent,
FinanciacionDialogComponent,
MatriculacionDialogComponent,
EntregarConfirmationPopup,
CursoDialogComponent,
ConfirmationDialogComponent,
SimulacionesComponent,
PresupuestoFinancieroComponent,
PresupuestoEconomicoComponent,
BalanceFinalComponent,
ProveedoresComponent,
ProveedorDetailComponent,
EstadoJuegosComponent,
FinanciacionesComponent,
CursosComponent,
EstadoJuegoDetailComponent,
PremiosDetailComponent,
RankingsComponent
],
imports: [
BrowserModule,
BrowserAnimationsModule,
FormsModule,
HttpClientModule,
MaterialModule,
ReactiveFormsModule,
AppRoutingModule,
HttpClientModule,
AngularFireModule.initializeApp(environment.firebase),
AngularFireAuthModule,
ChartsModule
],
providers: [
ProyectoService,
EscenariosService,
UsuarioService,
DecisionesService,
MessagesService,
ProveedoresService,
FinanciacionService
],
entryComponents: [RegisterComponent, OpcionDialogComponent, ConsecuenciaDialogComponent,
FinanciacionDialogComponent, CursoDialogComponent, MatriculacionDialogComponent, EntregarConfirmationPopup, ConfirmationDialogComponent],
bootstrap: [AppComponent, HomeComponent]
})
export class AppModule { }
//platformBrowserDynamic().bootstrapModule(AppModule);<file_sep>/src/app/decisiones.service.spec.ts
import { TestBed } from '@angular/core/testing';
import { DecisionesService } from './decisiones.service';
describe('DecisionesService', () => {
beforeEach(() => TestBed.configureTestingModule({}));
it('should be created', () => {
const service: DecisionesService = TestBed.get(DecisionesService);
expect(service).toBeTruthy();
});
});
<file_sep>/src/app/login/components/register.component.ts
import { Component, OnInit } from '@angular/core';
import { AngularFireAuth } from '@angular/fire/auth';
import * as firebase from 'firebase/app';
import { MatDialogRef, MatSnackBar } from '@angular/material';
import { FormControl, Validators, FormGroup, AbstractControl, ValidationErrors } from '@angular/forms';
import { Router } from '@angular/router';
@Component({
selector: 'register-dialog',
templateUrl: './register.component.html',
styleUrls: ['./register.component.css']
})
export class RegisterComponent implements OnInit {
email = new FormControl('', [Validators.required, Validators.email])
password = new FormControl('', [Validators.required])
repeatPassword = new FormControl('', [Validators.required])
registerForm: FormGroup = new FormGroup({
email: this.email,
password: <PASSWORD>,
repeatPassword: this.<PASSWORD>
}, this.validatePassword );
constructor(public dialogRef: MatDialogRef<RegisterComponent>,
private afAuth: AngularFireAuth,
private router: Router,
public snackBar: MatSnackBar) { }
ngOnInit() {
}
onRegister() {
if (this.registerForm.valid) {
this.afAuth.auth.createUserWithEmailAndPassword(this.email.value, this.password.value)
.then(_ => this.afAuth.auth.signInWithEmailAndPassword(this.email.value, this.password.value)
.then(_ => {
this.dialogRef.close();
this.router.navigate(['/home']);
})
.catch(e => this.openSnackBar(e.message)))
.catch(e => this.openSnackBar(e.message));
}
}
validatePassword(c: AbstractControl): ValidationErrors {
var error = { passwordNoMatch: true };
var password = c.get('password');
var repeat = c.get('repeatPassword');
if (password.value != repeat.value){
repeat.setErrors(error)
return { passwordNoMatch: true };
}else{
repeat.setErrors(null);
return null;
}
}
onClose() {
this.dialogRef.close();
}
openSnackBar(message: string, action: string = "OK") {
this.snackBar.open(message, action, {
duration: 2000,
//extraClasses: ['error-snack-bar']
});
}
}
<file_sep>/src/app/escenario-detalle/escenario-detalle.component.ts
import { Component, OnInit, ViewChild, ElementRef } from '@angular/core';
import { COMMA, ENTER } from '@angular/cdk/keycodes';
import { Validators, FormControl, FormGroup } from '@angular/forms';
import { ActivatedRoute, Router } from '@angular/router';
import { EscenariosService } from '../escenarios.service';
import { MessagesService } from '../messages.service';
import { CursosService } from '../cursos.service';
import { MatAutocompleteSelectedEvent, MatAutocomplete } from '@angular/material';
import { startWith, map } from 'rxjs/operators';
import { of, Observable } from 'rxjs';
import { UsuarioService } from '../usuario.service';
@Component({
selector: 'app-escenario-detalle',
templateUrl: './escenario-detalle.component.html',
styleUrls: ['./escenario-detalle.component.css']
})
export class EscenarioDetalleComponent implements OnInit {
separatorKeysCodes: number[] = [ENTER, COMMA];
filtredCursos: Observable<string[]>;
usuario;
allCursos;
configuracionMercado;
escenario = {
id: null,
titulo: '',
maximosPeriodos: null,
nombrePeriodos: 'Mes',
descripcion: '',
impuestoPorcentaje: null,
balanceInicial: {
activo: {
caja: 0,
cuentasPorCobrar: 0,
cuentasPorCobrarPeriodos: 0,
inventario: 0,
maquinaria: 0,
amortizacionAcumulada: 0
},
pasivo: {
proveedores: 0,
proveedoresPeriodos: 0,
deudasBancarias: 0,
deudasBancariasPeriodos: 0
},
patrimonioNeto: {
capitalSocial: 0,
resultadoDelEjercicio: 0
}
},
estadoInicial: {
costoFijo: 0,
costoVariable: 0,
stock: 0,
produccionMensual: 0,
calidad: 0,
publicidad: 0,
cantidadVendedores: 0
},
cursos: [],
invalid: null
};
descripcion = new FormControl('', [Validators.required]);
titulo = new FormControl('', [Validators.required]);
maximosPeriodos = new FormControl(new Number(), [Validators.required, Validators.min(1)]);
nombrePeriodos = new FormControl({ value: '', disabled: true }, [Validators.required]);
impuestoPorcentaje = new FormControl(new Number(), [Validators.required, Validators.min(0), Validators.max(99.9)]);
cursos = new FormControl();
escenarioForm: FormGroup = new FormGroup({
descripcion: this.descripcion,
titulo: this.titulo,
maximosPeriodos: this.maximosPeriodos,
nombrePeriodos: this.nombrePeriodos,
cursos: this.cursos
});
caja = new FormControl(new Number(), [Validators.required, Validators.min(0)]);
cuentasPorCobrar = new FormControl(new Number(), [Validators.required, Validators.min(0)]);
cuentasPorCobrarPeriodos = new FormControl(new Number(), [Validators.required, Validators.min(0)]);
inventario = new FormControl(new Number(), [Validators.required, Validators.min(0)]);
maquinaria = new FormControl(new Number(), [Validators.required, Validators.min(0)]);
amortizacionAcumulada = new FormControl(new Number(), [Validators.required, Validators.min(0)]);
activoForm: FormGroup = new FormGroup({
caja: this.caja,
cuentasPorCobrar: this.cuentasPorCobrar,
inventario: this.inventario,
maquinaria: this.maquinaria,
amortizacionAcumulada: this.amortizacionAcumulada
});
proveedores = new FormControl(new Number(), [Validators.required, Validators.min(0)]);
proveedoresPeriodos = new FormControl(new Number(), [Validators.required, Validators.min(0)]);
deudasBancarias = new FormControl(new Number(), [Validators.required, Validators.min(0)]);
deudasBancariasPeriodos = new FormControl(new Number(), [Validators.required, Validators.min(0)]);
pasivoForm: FormGroup = new FormGroup({
proveedores: this.proveedores,
proveedoresPeriodos: this.proveedoresPeriodos,
deudasBancarias: this.deudasBancarias,
deudasBancariasPeriodos: this.deudasBancariasPeriodos
});
capitalSocial = new FormControl(new Number(), [Validators.required, Validators.min(0)]);
resultadoDelEjercicio = new FormControl(new Number(), [Validators.required, Validators.min(0)]);
patrimonioNetoForm: FormGroup = new FormGroup({
capitalSocial: this.capitalSocial,
resultadoDelEjercicio: this.resultadoDelEjercicio
});
calidad = new FormControl(new Number(), [Validators.required, Validators.min(0)]);
costoFijo = new FormControl(new Number(), [Validators.required, Validators.min(0)]);
costoVariable = new FormControl(new Number(), [Validators.required, Validators.min(0)]);
produccionMensual = new FormControl(new Number(), [Validators.required, Validators.min(0)]);
stock = new FormControl(new Number(), [Validators.required, Validators.min(0)]);
publicidad = new FormControl(new Number(), [Validators.required, Validators.min(0)]);
cantidadVendedores = new FormControl(new Number(), [Validators.required, Validators.min(0)]);
estadoInicialForm: FormGroup = new FormGroup({
calidad: this.calidad,
costoFijo: this.costoFijo,
costoVariable: this.costoVariable,
produccionMensual: this.produccionMensual,
stock: this.stock,
publicidad: this.publicidad,
cantidadVendedores: this.cantidadVendedores
});
forecasts = [{
proyectoId: 1,
periodo: 0,
cantidadUnidades: 0,
precio: 0
}, {
proyectoId: 1,
periodo: 1,
cantidadUnidades: 0,
precio: 0
}, {
proyectoId: 1,
periodo: 2,
cantidadUnidades: 0,
precio: 0
}, {
proyectoId: 1,
periodo: 3,
cantidadUnidades: 0,
precio: 0
}]
porcentajeCaja = new FormControl('', [Validators.required]);
porcentajeVentas = new FormControl('', [Validators.required]);
porcentajeRenta = new FormControl('', [Validators.required]);
porcentajeEscenario = new FormControl('', [Validators.required, Validators.min(0), Validators.max(100)]);
puntajeForm: FormGroup = new FormGroup({
porcentajeCaja: this.porcentajeCaja,
porcentajeVentas: this.porcentajeVentas,
porcentajeRenta: this.porcentajeRenta,
porcentajeEscenario: this.porcentajeEscenario
});
hasProyectos = false;
constructor(private escenariosService: EscenariosService,
private usuariosService: UsuarioService,
private route: ActivatedRoute,
private router: Router,
private cursosService: CursosService,
private messageService: MessagesService) {
}
puntajes = {
escenarioId: null,
porcentajeCaja: 0,
porcentajeVentas: 0,
porcentajeRenta: 0,
porcentajeEscenario: 0
}
@ViewChild('cursosInput', { static: false }) cursosInput: ElementRef<HTMLInputElement>;
@ViewChild('auto', { static: false }) matAutocomplete: MatAutocomplete;
ngOnInit() {
var id = Number(this.route.snapshot.paramMap.get('id'));
if (id) {
this.getEscenario(id).subscribe(escenario => {
this.escenario = escenario
this.getConfiguracionMercado(escenario)
this.getHasProyectos(escenario.id);
this.escenariosService.getCursosEscenario(id).subscribe(cursosEscenario => {
this.escenario.cursos = cursosEscenario;
})
});
this.getPuntajes(id);
}
this.getCursos();
}
getHasProyectos(escenarioId) {
return this.escenariosService.getProyectosByEscenario(escenarioId).subscribe(proyectos => {
this.hasProyectos = (proyectos.length > 0)
})
}
getPuntajes(escenarioId) {
this.escenariosService.getPuntajes(escenarioId).subscribe(puntajes => {
if (puntajes) {
this.puntajes = puntajes;
} else {
this.puntajes.escenarioId = escenarioId;
}
})
}
getCursos() {
this.cursosService.getCursos().subscribe(cursos => {
this.allCursos = cursos;
this.filtredCursos = of(cursos);
this.filtredCursos = this.cursos.valueChanges.pipe(
startWith(null),
map((curso: string | null) => {
return curso ? this._filterCurso(curso) : this.allCursos.slice()
}));
})
}
selectedCurso(event: MatAutocompleteSelectedEvent) {
this.escenario.cursos.push(
event.option.value
);
this.cursosInput.nativeElement.value = '';
this.cursos.setValue(null);
}
removeCurso(curso): void {
const index = this.escenario.cursos.indexOf(curso);
if (index >= 0) {
this.escenario.cursos.splice(index, 1);
}
}
private _filterCurso(value: string): string[] {
if (value.toLowerCase) {
const filterValue = value.toLowerCase();
return this.allCursos.filter(curso => curso.nombre.toLowerCase().indexOf(filterValue) === 0);
} else {
return this.allCursos;
}
}
getEscenario(id) {
return this.escenariosService.getEscenario(id);
}
getPeriodosArray() {
var maximosPeriodos = Number(this.escenario.maximosPeriodos);
return [...Array(maximosPeriodos).keys(), maximosPeriodos];
}
save() {
if (this.escenarioForm.valid && this.activoForm.valid && this.pasivoForm.valid && this.patrimonioNetoForm.valid
&& this.puntajeForm.valid) {
if (this._porcentajesValidos()) {
this.escenariosService[this.escenario.id ? 'modifyEscenario' : 'createEscenario'](this.escenario)
.subscribe(escenario => {
this.escenariosService.updateCursosEscenario(escenario.id, this.escenario.cursos).subscribe(_ => {
this.escenariosService.postPuntajes(escenario.id, this.puntajes).subscribe(_ => {
if (this.escenario.id) {
const error = this._getErroresConfiguracionesMercado();
if (error) {
this.messageService.openSnackBar(error)
} else {
this.escenariosService.postConfiguracionesMercado(this.configuracionMercado).subscribe(_ => {
this.messageService.openSnackBar("Escenario modificado");
this.router.navigate(['/escenarios'])
})
}
} else {
this.messageService.openSnackBar("Escenario modificado");
this.router.navigate(['/escenarios/' + escenario.id])
}
})
})
});
}
} else {
this.messageService.openSnackBar("ERROR: Revise los datos ingresados.");
}
}
getConfiguracionMercado(escenario) {
this.escenariosService.getConfiguracionMercado(escenario.id).subscribe(configuracionMercado => {
if (configuracionMercado.restriccionPrecio) {
this.configuracionMercado = configuracionMercado;
} else {
this.setConfiguracionMercado(escenario)
}
})
}
getMercadosPeriodo() {
if (this.escenario.maximosPeriodos !== this.configuracionMercado.mercadosPeriodo.length && this.escenario.maximosPeriodos !== null) {
this.setConfiguracionMercado(this.escenario);
}
return this.configuracionMercado.mercadosPeriodo;
}
setConfiguracionMercado(escenario) {
const arrayPeriodos = [...Array(escenario.maximosPeriodos).keys()],
array3 = [...Array(3).keys()],
array4 = [...Array(4).keys()];
this.configuracionMercado = {
empresasCompetidoras: array3.map((_, index) => {
return {
escenarioId: escenario.id,
nombre: 'Empresa ' + index,
bajo: 0,
medio: 0,
alto: 0
}
}),
mercadosPeriodo: arrayPeriodos.map((_, index) => {
return {
escenarioId: escenario.id,
periodo: index + 1,
bajo: 0,
medio: 0,
alto: 0
}
}),
ponderacionesMercado: {
precioDesde: array3.map((_, index) => {
return {
escenarioId: escenario.id,
concepto: "PRECIO_DESDE",
valor: 0,
bajo: 0,
medio: 0,
alto: 0
}
}),
vendedoresDesde: array3.map((_, index) => {
return {
escenarioId: escenario.id,
concepto: "VENDEDORES_DESDE",
valor: 0,
bajo: 0,
medio: 0,
alto: 0
}
}),
publicidadDesde: array3.map((_, index) => {
return {
escenarioId: escenario.id,
concepto: "PUBLICIDAD_DESDE",
valor: 0,
bajo: 0,
medio: 0,
alto: 0
}
}),
modalidadCobro: array4.map((_, index) => {
return {
escenarioId: escenario.id,
concepto: "MODALIDAD_DE_COBRO",
valor: index,
bajo: 0,
medio: 0,
alto: 0
}
}),
calidadDesde: array3.map((_, index) => {
return {
escenarioId: escenario.id,
concepto: "CALIDAD_DESDE",
valor: 0,
bajo: 0,
medio: 0,
alto: 0
}
})
},
restriccionPrecio: {
escenarioId: escenario.id,
precioMin: 0,
precioMax: 0
}
}
}
getModalidadCobroDesc(modCobroIndex) {
return ["Contado", "a 30 dias", "a 60 dias", "a 90 dias"][modCobroIndex]
}
_getErroresConfiguracionesMercado() {
return [this._validateObligatory(this.configuracionMercado.empresasCompetidoras, "nombre"),
this._validateObligatory(this.configuracionMercado.mercadosPeriodo, null),
this._validateObligatory(this.configuracionMercado.ponderacionesMercado.precioDesde, "valor"),
this._validateSecuencia(this.configuracionMercado.ponderacionesMercado.precioDesde, "valor"),
this._validatePrecioContraIntervalo(),
this._validateObligatory(this.configuracionMercado.ponderacionesMercado.modalidadCobro, "valor"),
this._validateSecuencia(this.configuracionMercado.ponderacionesMercado.modalidadCobro, "valor"),
this._validateObligatory(this.configuracionMercado.ponderacionesMercado.publicidadDesde, "valor"),
this._validateSecuencia(this.configuracionMercado.ponderacionesMercado.publicidadDesde, "valor"),
this._validateObligatory(this.configuracionMercado.ponderacionesMercado.calidadDesde, "valor"),
this._validateSecuencia(this.configuracionMercado.ponderacionesMercado.calidadDesde, "valor"),
this._validateObligatory(this.configuracionMercado.ponderacionesMercado.vendedoresDesde, "valor"),
this._validateSecuencia(this.configuracionMercado.ponderacionesMercado.vendedoresDesde, "valor"),
this._validateIntervaloPrecio()].find(elem => elem !== true)
}
_validateSecuencia(array, prop) {
var arrayValor = array.map(elem => elem[prop]),
arrayOrdernado = arrayValor.concat([]).sort((a, b) => a > b ? 1 : -1);
if (JSON.stringify(arrayOrdernado) !== JSON.stringify(arrayValor)) {
return "Revise las secuencias de valores en las configuraciones de mercado"
}
return true;
}
_validateObligatory(array, additionalProp) {
return array.find(elem => {
return (!(elem.bajo >= 0 && elem.medio >= 0 && elem.alto >= 0) ||
(additionalProp ? !(elem[additionalProp] || elem[additionalProp] === 0) : false))
}) ? "Falta completar datos en la configuracion del mercado" : true;
}
_validatePrecioContraIntervalo() {
return this.configuracionMercado.ponderacionesMercado.precioDesde.find(elem => {
return (elem.valor > this.configuracionMercado.restriccionPrecio.precioMax ||
elem.valor < this.configuracionMercado.restriccionPrecio.precioMin)
}) ? "El rango de precios y los precios ingresados no coinciden" : true;
}
_validateIntervaloPrecio() {
return (this.configuracionMercado.restriccionPrecio.precioMin > 0 &&
this.configuracionMercado.restriccionPrecio.precioMax > 0 &&
this.configuracionMercado.restriccionPrecio.precioMin < this.configuracionMercado.restriccionPrecio.precioMax)
? true : "Ingrese un Intervalo de Precio valido"
}
_porcentajesValidos() {
if (this.puntajes.porcentajeCaja + this.puntajes.porcentajeVentas + this.puntajes.porcentajeRenta !== 100) {
this.messageService.openSnackBar("Puntajes: Los porcentajes ingresados para caja, renta y ventas deben sumar 100%")
return false;
}
return true;
}
}
<file_sep>/src/app/financiaciones/financiaciones.component.ts
import { Component, OnInit, Input } from '@angular/core';
import { EscenariosService } from '../escenarios.service';
import { FinanciacionService } from '../financiacion.service';
import { MatDialog } from '@angular/material';
import { FinanciacionDialogComponent } from './financiacion-dialog/financiacion-dialog.component';
@Component({
selector: 'app-financiaciones',
templateUrl: './financiaciones.component.html',
styleUrls: ['./financiaciones.component.css']
})
export class FinanciacionesComponent implements OnInit {
@Input() escenario: any;
@Input() hasProyectos: boolean;
financiaciones;
constructor(private dialog: MatDialog,
private escenarioService: EscenariosService,
private financiacionService: FinanciacionService) { }
ngOnInit() {
this.getFinanciaciones();
}
getFinanciaciones() {
this.escenarioService.getFinanciaciones(this.escenario.id).subscribe(financiaciones => this.financiaciones = financiaciones);
}
editFinanciacion(financiacion) {
const dialogRef = this.dialog.open(FinanciacionDialogComponent, {
width: '400px',
data: financiacion
});
dialogRef.afterClosed().subscribe(_ => this.getFinanciaciones());
}
removeFinanciacion(financiacion) {
this.financiacionService.removeFinanciacion(financiacion).subscribe(_ => this.getFinanciaciones())
}
createFinanciacion(){
const dialogRef = this.dialog.open(FinanciacionDialogComponent, {
width: '400px',
data: {
escenarioId: this.escenario.id,
descripcion:"",
tea: 0,
cantidadCuotas: 0
}
});
dialogRef.afterClosed().subscribe(_ => this.getFinanciaciones());
}
}
<file_sep>/src/app/matriculacion-dialog/matriculacion-dialog.component.ts
import { Component, OnInit, Inject } from '@angular/core';
import { MatDialogRef, MAT_DIALOG_DATA } from '@angular/material';
import { FormControl, Validators, FormGroup } from '@angular/forms';
import { MessagesService } from '../messages.service';
import { UsuarioService } from '../usuario.service';
@Component({
selector: 'matriculacion-dialog',
templateUrl: './matriculacion-dialog.component.html',
styleUrls: ['./matriculacion-dialog.component.css']
})
export class MatriculacionDialogComponent implements OnInit {
curso;
usuario;
nombre = new FormControl('', [Validators.required])
clave = new FormControl('', [Validators.required])
cursoForm: FormGroup = new FormGroup({
nombre: this.nombre,
clave: this.clave
});
constructor(public dialogRef: MatDialogRef<MatriculacionDialogComponent>,
@Inject(MAT_DIALOG_DATA) public data, private usuarioService: UsuarioService,
private messageService: MessagesService) { }
ngOnInit() {
this.usuario = JSON.parse(JSON.stringify(this.data));
this.curso = {
nombre: "",
clave: ""
};
}
cancel() {
this.dialogRef.close();
}
matricular() {
if (this.cursoForm.valid) {
let cursoSend = {nombre: this.curso.nombre, clave: btoa(this.curso.clave)};
this.usuarioService.matricular(this.usuario, cursoSend).subscribe(_ => {
this.dialogRef.close();
window.location.reload();
});
}
}
}
<file_sep>/src/app/proveedores/proveedores.component.ts
import { Component, OnInit, Input } from '@angular/core';
import { EscenariosService } from '../escenarios.service';
import { ProveedoresService } from '../proveedores.service';
@Component({
selector: 'app-proveedores',
templateUrl: './proveedores.component.html',
styleUrls: ['./proveedores.component.css']
})
export class ProveedoresComponent implements OnInit {
@Input() escenario: any;
@Input() hasProyectos: boolean;
proveedores;
constructor(private escenariosService: EscenariosService,
private proveedoresService: ProveedoresService) { }
ngOnInit() {
this.getProveedores();
}
getProveedores() {
this.escenariosService.getProveedores(this.escenario.id).subscribe(proveedores => {
this.proveedores = proveedores;
});
}
removeProveedor(proveedor) {
this.proveedoresService.removeProveedor(proveedor).subscribe(_ => this.getProveedores());
}
}
<file_sep>/README.md
# utn-simulador-ui
1. Run npm install
2. Run ng serve
3. Go to localhost:4200
<file_sep>/src/app/flujo-fondos/flujo-fondos.component.ts
import { Component, OnInit, Input } from '@angular/core';
import { ProyectoService } from '../proyecto.service';
import { trigger, state, style, transition, animate } from '@angular/animations';
import { ActivatedRoute } from '@angular/router';
import { UsuarioService } from '../usuario.service';
@Component({
selector: 'app-flujo-fondos',
templateUrl: './flujo-fondos.component.html',
styleUrls: ['./flujo-fondos.component.css'],
animations: [
trigger('detailExpand', [
state('collapsed', style({ height: '0px', minHeight: '0' })),
state('expanded', style({ height: '*' })),
transition('expanded <=> collapsed', animate('225ms cubic-bezier(0.4, 0.0, 0.2, 1)')),
]),
]
})
export class FlujoFondosComponent implements OnInit {
@Input() proyecto: any;
@Input() forecast: boolean;
flujoFondos;
periodos;
escenario;
constructor(private proyectoService: ProyectoService) { }
ngOnInit() {
this.getPeriodos(this.proyecto.id);
this.getFlujoFondos(this.proyecto.id);
this.escenario = this.proyecto.escenario;
}
getFlujoFondos(proyectoId) {
this.proyectoService.getFlujoFondos(proyectoId, this.forecast).subscribe(ff => {
this.flujoFondos = ff;
})
}
getPeriodos(proyectoId) {
this.proyectoService.getPeriodoActual(proyectoId, this.forecast).subscribe(periodoActual => {
this.periodos = [...Array(periodoActual).keys(), periodoActual];
})
}
getCuentaPeriodo(cuentasPeriodo, periodo, defaultCero = true, signoInvertido = false) {
var periodo = cuentasPeriodo && cuentasPeriodo.find(cuentaPeriodo => cuentaPeriodo.periodo === periodo)
var montoCrudo = periodo ? periodo.monto : (defaultCero ? 0 : "");
montoCrudo = signoInvertido ? -montoCrudo : montoCrudo;
if (montoCrudo < 0) {
return "(" + Math.abs(montoCrudo).toLocaleString() + ")";
} else if (montoCrudo === 0) {
return montoCrudo;
} else {
return montoCrudo.toLocaleString();
}
}
}
<file_sep>/src/app/toma-decisiones/toma-decisiones.component.ts
import { Component, OnInit, EventEmitter } from '@angular/core';
import { ProyectoService } from '../proyecto.service';
import { Router, ActivatedRoute } from '@angular/router';
import { MessagesService } from '../messages.service';
import { EscenariosService } from '../escenarios.service';
import { UsuarioService } from '../usuario.service';
import { MatRadioChange } from '@angular/material';
@Component({
selector: 'app-toma-decisiones',
templateUrl: './toma-decisiones.component.html',
styleUrls: ['./toma-decisiones.component.css']
})
export class TomaDecisionesComponent implements OnInit {
decisiones: Array<any> = [];
escenario;
estado;
proyecto;
modalidadCobro: Array<any> = [];
forecasts: Array<any> = [];
proveedores: Array<any> = [];
proveedorSeleccionado;
usuario;
financiaciones;
credito;
totalActivo = 0;
totalPasivoPatrimonioNeto = 0;
constructor(
private proyectoService: ProyectoService,
private escenarioService: EscenariosService,
private usuarioService: UsuarioService,
private router: Router,
private route: ActivatedRoute,
private messageService: MessagesService) { }
ngOnInit() {
var escenarioId = Number(this.route.snapshot.paramMap.get('escenarioId'));
this.getProyecto(escenarioId, this.usuarioService.usuario.id);
this.getFinanciaciones(escenarioId);
}
getEscenario(escenarioId) {
this.escenarioService.getEscenario(escenarioId).subscribe(escenario => this.escenario = escenario);
}
getFinanciaciones(escenarioId) {
this.escenarioService.getFinanciaciones(escenarioId).subscribe(financiaciones => this.financiaciones = financiaciones);
}
getProyecto(escenarioId, usuarioId) {
this.usuarioService.getProyecto(escenarioId, usuarioId).subscribe(estado => {
this.estado = estado;
this.proyecto = estado.proyecto;
this.escenario = estado.proyecto.escenario;
this.getTotalActivo();
this.getTotalPasivoPatrimonioNeto();
this.getProveedores(this.proyecto.id)
this.getCredito(this.proyecto.id)
this.getDecisiones(this.proyecto.id)
this.buildModalidadDeCobro(this.proyecto.id, estado.proyecto.modalidadCobro);
this.buildForecast(this.proyecto.id);
});
}
getCredito(proyectoId) {
this.proyectoService.getCredito(proyectoId)
.subscribe(credito => this.credito = credito || {
monto: 0,
periodoInicial: 0,
proyectoId: this.proyecto.id
});
}
getDecisiones(proyectoId) {
this.proyectoService.getDecisiones(proyectoId)
.subscribe(decisiones => this.decisiones = decisiones);
}
getProveedores(proyectoId) {
this.proyectoService.getProveedores(proyectoId).subscribe(proveedores => {
this.proveedores = proveedores;
var proveedorSeleccionado = proveedores.find(p => p.seleccionado)
if (proveedorSeleccionado) {
this.proveedorSeleccionado = proveedorSeleccionado.id;
}
})
}
buildForecast(proyectoId) {
this.proyectoService.getForecast(proyectoId).subscribe(forecasts => {
var periodos = this.estado.proyecto.escenario.maximosPeriodos;
if(forecasts.length > 0 && forecasts.length >= periodos + 1) {
this.forecasts = forecasts.slice(0, periodos + 1)
} else {
var periodosFaltantes = [...Array(periodos - forecasts.length).keys(), periodos - forecasts.length].map(periodo => {
return {
proyectoId: proyectoId,
periodo: forecasts.length + periodo,
cantidadUnidades: 0,
precio: 0
}
});
this.forecasts = this.forecasts.concat(forecasts);
this.forecasts = this.forecasts.concat(periodosFaltantes);
}
})
}
buildModalidadDeCobro(proyectoId, modalidadCobro) {
var modalidadCobroTemplate = [{
proyectoId: this.proyecto.id,
offsetPeriodo: 0,
porcentaje: 0
}, {
proyectoId: this.proyecto.id,
offsetPeriodo: 1,
porcentaje: 0
}, {
proyectoId: this.proyecto.id,
offsetPeriodo: 2,
porcentaje: 0
}, {
proyectoId: this.proyecto.id,
offsetPeriodo: 3,
porcentaje: 0
}];
if (modalidadCobro) {
this.modalidadCobro = modalidadCobroTemplate.map(mct => {
return { ...mct, ...modalidadCobro.find(mc => mc.offsetPeriodo === mct.offsetPeriodo) };
});
} else {
this.modalidadCobro = modalidadCobroTemplate;
}
}
getModalidadDePagoParaPeriodo(modalidadPago, periodo) {
if (modalidadPago) {
var modalidadDePagoParaPeriodo = modalidadPago.find(mp => mp.offsetPeriodo === periodo);
}
return (modalidadDePagoParaPeriodo && modalidadDePagoParaPeriodo.porcentaje > 0) ?
`${modalidadDePagoParaPeriodo.porcentaje}%` : "";
}
getModalidadDeCobroDescr(offsetPeriodo) {
return {
0: "Contado",
1: "a 30 Dias",
2: "a 60 Dias",
3: "a 90 Dias"
}[offsetPeriodo];
}
getCantidadDecisionesTomadas() {
return this.decisiones ? this.decisiones.filter(d => d.opcionTomada).length : 0;
}
getOpcionesTomadas() {
return this.decisiones.map(d => d.opciones.find(o => o.id === d.opcionTomada)).filter(d => d);
}
getAumentoCostoFijo() {
var aumentoCostoFijo = 0;
this.getOpcionesTomadas().forEach(o => aumentoCostoFijo = aumentoCostoFijo + o.variacionCostoFijo);
return aumentoCostoFijo != 0 ? (aumentoCostoFijo % 1 != 0 ? aumentoCostoFijo.toFixed(2) : aumentoCostoFijo) : null;
}
getAumentoCostoVariable() {
var aumentoCostoVariable = this.getProveedorSeleccionado() ? this.getProveedorSeleccionado().variacionCostoVariable : 0;
this.getOpcionesTomadas().forEach(o => aumentoCostoVariable = aumentoCostoVariable + o.variacionCostoVariable);
return aumentoCostoVariable != 0 ? (aumentoCostoVariable % 1 != 0 ? aumentoCostoVariable.toFixed(2) : aumentoCostoVariable) : null;
}
getAumentoProduccionMensual() {
var aumentoProduccionMensual = 0;
this.getOpcionesTomadas().forEach(o => aumentoProduccionMensual = aumentoProduccionMensual + o.variacionProduccion);
return aumentoProduccionMensual != 0 ? aumentoProduccionMensual : null;
}
getAumentoCalidad() {
var aumentoCalidad = this.getProveedorSeleccionado() ? this.getProveedorSeleccionado().variacionCalidad : 0;
this.getOpcionesTomadas().forEach(o => aumentoCalidad = aumentoCalidad + o.variacionCalidad);
return aumentoCalidad != 0 ? aumentoCalidad : null;
}
getAumentoPublicidad() {
var aumentoPublicidad = 0;
this.getOpcionesTomadas().forEach(o => aumentoPublicidad = aumentoPublicidad + o.variacionPublicidad);
return aumentoPublicidad != 0 ? aumentoPublicidad : null;
}
getAumentoCantidadVendedores() {
var aumentoCantidadVendedores = 0;
this.getOpcionesTomadas().forEach(o => aumentoCantidadVendedores = aumentoCantidadVendedores + o.variacionCantidadVendedores);
return aumentoCantidadVendedores != 0 ? aumentoCantidadVendedores : null;
}
simular() {
if (this.inputsValidos()) {
//Grabar FORECAST
this.proyectoService.forecast(this.proyecto.id, this.forecasts)
.subscribe(_ => {
//Grabar MODALIDAD COBRO
this.proyectoService.modalidadCobro(this.proyecto.id, this.modalidadCobro).subscribe(_ => {
//Grabar PROVEEDORES
this.proyectoService.proveedorSeleccionado(this.proyecto.id, this.proveedorSeleccionado).subscribe(_ => {
//Grabar Credito
this.proyectoService.tomarCredito(this.credito).subscribe(_ => {
//SIMULAR
this.proyectoService.simular(this.proyecto.id, this.getOpcionesTomadas())
.subscribe(_ => this.router.navigateByUrl(`/simulaciones/escenario/${this.escenario.id}/resultados`))
})
})
})
});
}
}
_simular() {
}
getEvenNumber = function(num) {
return Math.floor(Number(num)/2) > 0 ? new Array(Math.floor(Number(num)/2)) : new Array(0);
}
getOddNumber = function(num) {
return Number(num) % 2 != 0;
}
getTotalActivo() {
this.totalActivo = this.escenario.balanceInicial.activo.caja + this.escenario.balanceInicial.activo.cuentasPorCobrar + this.escenario.balanceInicial.activo.inventario + this.escenario.balanceInicial.activo.maquinaria + this.escenario.balanceInicial.activo.amortizacionAcumulada;
}
getTotalPasivoPatrimonioNeto() {
this.totalPasivoPatrimonioNeto = this.escenario.balanceInicial.pasivo.proveedores + this.escenario.balanceInicial.pasivo.deudasBancarias + this.escenario.balanceInicial.patrimonioNeto.capitalSocial + this.escenario.balanceInicial.patrimonioNeto.resultadoDelEjercicio;
}
inputsValidos() {
//Validar si se tomaron todas las opciones
var opcionesTomadas = this.getOpcionesTomadas();
if (opcionesTomadas.length !== this.decisiones.length) {
this.messageService.openSnackBar("Toma todas las decisiones antes de simular!");
return false;
}
//Validar si el porcentaje de cobro es 100% en total entre todos los periodos
var modalidadCobroPorcentajeTotal = this.modalidadCobro.filter(elem => elem.porcentaje > 0)
.reduce((acum, elem) => acum + elem.porcentaje, 0)
if (modalidadCobroPorcentajeTotal !== 100) {
this.messageService.openSnackBar("Los porcentajes en su modalidad de cobro deben sumar 100%!");
return false;
}
//Validar si los valores de forecast de venta son correctos
var forecastNegativos = this.forecasts.filter(forecast => forecast.cantidadUnidades !== null && forecast.cantidadUnidades < 0)
if (forecastNegativos.length > 0) {
this.messageService.openSnackBar("Todas las cantidades vendidas del forecast de venta deben ser numeros positivos");
return false;
}
//Validar Proveedor seleccionado
if (!this.proveedorSeleccionado) {
this.messageService.openSnackBar("Por favor seleccione un proveedor");
return false;
}
//Validar Credito: Valores negaticos
if (this.credito.monto < 0 || this.credito.periodoInicial < 0) {
this.messageService.openSnackBar("El monto de financiacion y el periodo inicial deben ser numeros positivos");
return false;
}
//Validar Credito seleccionado
if (this.credito.monto > 0 && !this.credito.financiacionId) {
this.messageService.openSnackBar("Usted ha dispuesto un monto de financiacion. Por favor seleccione un tipo de credito");
return false;
}
//Validar Credito periodo valido
if (this.credito.monto > 0 && this.credito.periodoInicial > this.escenario.maximosPeriodos) {
this.messageService.openSnackBar("El Periodo Inicial del credito no puede ser mayor a los periodos del ejercicio");
return false;
}
return true;
}
onSeleccionarProveedor(proveedor) {
this.proveedorSeleccionado = proveedor.id;
}
onSeleccionarFinanciacion(financiacion) {
this.credito.financiacionId = financiacion.id;
}
getProveedorSeleccionado() {
return this.proveedores.find(p => p.id == this.proveedorSeleccionado);
}
}
<file_sep>/src/app/flujo-fondos/flujo-fondos.component.html
<table class="mat-table table" *ngIf="flujoFondos">
<tr class="mat-header-row">
<th class="mat-header-cell desc-column">Descripcion</th>
<th *ngFor="let periodo of periodos" class="mat-header-cell value-column"> {{escenario.nombrePeriodos}} {{periodo}} </th>
</tr>
<!--INGRESOS_AFECTOS_A_IMPUESTOS-->
<tr *ngIf="flujoFondos.INGRESOS_AFECTOS_A_IMPUESTOS as flujo" class="mat-row">
<td class="mat-cell">
<p class="flujo-column">
{{ flujo.descripcion }}
</p>
<p *ngFor="let cuenta of flujo.cuentas;" class="desc-cuenta">
{{ cuenta.descripcion }}
</p>
</td>
<td *ngFor="let periodo of periodos;" class="mat-cell value-column">
<p>
</p>
<p *ngFor="let cuenta of flujo.cuentas;">
{{ getCuentaPeriodo(cuenta.cuentasPeriodo,periodo,true, false) }}
</p>
</td>
</tr>
<!--EGRESOS_AFECTOS_A_IMPUESTOS-->
<tr *ngIf="flujoFondos.EGRESOS_AFECTOS_A_IMPUESTOS as flujo" class="mat-row">
<td class="mat-cell ">
<p class="flujo-column">
{{ flujo.descripcion }}
</p>
<p *ngFor="let cuenta of flujo.cuentas;" class="desc-cuenta">
{{ cuenta.descripcion }}
</p>
</td>
<td *ngFor="let periodo of periodos;" class="mat-cell value-column">
<p>
</p>
<p *ngFor="let cuenta of flujo.cuentas;">
{{ getCuentaPeriodo(cuenta.cuentasPeriodo,periodo,true, true, true) }}
</p>
</td>
</tr>
<!--GASTOS_NO_DESEMBOLSABLES-->
<tr *ngIf="flujoFondos.GASTOS_NO_DESEMBOLSABLES as flujo" class="mat-row">
<td class="mat-cell ">
<p class="flujo-column">
{{ flujo.descripcion }}
</p>
<p *ngFor="let cuenta of flujo.cuentas;">
{{ cuenta.descripcion }}
</p>
</td>
<td *ngFor="let periodo of periodos;" class="mat-cell value-column">
<p *ngFor="let cuenta of flujo.cuentas;">
{{ getCuentaPeriodo(cuenta.cuentasPeriodo,periodo,true, true) }}
</p>
</td>
</tr>
<!--UTILIDAD_ANTES_DE_IMPUESTOS-->
<tr *ngIf="flujoFondos.UTILIDAD_ANTES_DE_IMPUESTOS as flujo" class="mat-row">
<td class="mat-cell ">
<p class="flujo-column">
{{ flujo.descripcion }}
</p>
</td>
<td *ngFor="let periodo of periodos;" class="mat-cell value-column">
{{ getCuentaPeriodo(flujo.montosPeriodo,periodo,true, false) }}
</td>
</tr>
<!--IMPUESTOS-->
<tr *ngIf="flujoFondos.IMPUESTOS as flujo" class="mat-row">
<td class="mat-cell ">
<p class="flujo-column">
{{ flujo.descripcion }}
</p>
</td>
<td *ngFor="let periodo of periodos;" class="mat-cell value-column">
{{ getCuentaPeriodo(flujo.montosPeriodo,periodo,true, true) }}
</td>
</tr>
<!--UTILIDAD_DESPUES_DE_IMPUESTOS-->
<tr *ngIf="flujoFondos.UTILIDAD_DESPUES_DE_IMPUESTOS as flujo" class="mat-row">
<td class="mat-cell ">
<p class="flujo-column">
{{ flujo.descripcion }}
</p>
</td>
<td *ngFor="let periodo of periodos;" class="mat-cell value-column">
{{ getCuentaPeriodo(flujo.montosPeriodo,periodo,true, false) }}
</td>
</tr>
<!--AJUSTE_DE_GASTOS_NO_DESEMBOLSABLES-->
<tr *ngIf="flujoFondos.AJUSTE_DE_GASTOS_NO_DESEMBOLSABLES as flujo" class="mat-row">
<td class="mat-cell ">
<p class="flujo-column">
{{ flujo.descripcion }}
</p>
<p *ngFor="let cuenta of flujo.cuentas;">
{{ cuenta.descripcion }}
</p>
</td>
<td *ngFor="let periodo of periodos;" class="mat-cell value-column">
<p *ngFor="let cuenta of flujo.cuentas;">
{{ getCuentaPeriodo(cuenta.cuentasPeriodo,periodo,true, false) }}
</p>
</td>
</tr>
<!--INGRESOS_NO_AFECTOS_A_IMPUESTOS-->
<tr *ngIf="flujoFondos.INGRESOS_NO_AFECTOS_A_IMPUESTOS as flujo" class="mat-row">
<td class="mat-cell ">
<p class="flujo-column">
{{ flujo.descripcion }}
</p>
<p *ngFor="let cuenta of flujo.cuentas;" class="desc-cuenta">
{{ cuenta.descripcion }}
</p>
</td>
<td *ngFor="let periodo of periodos;" class="mat-cell value-column">
<p>
</p>
<p *ngFor="let cuenta of flujo.cuentas;">
{{ getCuentaPeriodo(cuenta.cuentasPeriodo,periodo,true, false) }}
</p>
</td>
</tr>
<!--EGRESOS_NO_AFECTOS_A_IMPUESTOS-->
<tr *ngIf="flujoFondos.EGRESOS_NO_AFECTOS_A_IMPUESTOS as flujo" class="mat-row">
<td class="mat-cell ">
<p class="flujo-column">
{{ flujo.descripcion }}
</p>
<p *ngFor="let cuenta of flujo.cuentas;" class="desc-cuenta">
{{ cuenta.descripcion }}
</p>
</td>
<td *ngFor="let periodo of periodos;" class="mat-cell value-column">
<p>
</p>
<p *ngFor="let cuenta of flujo.cuentas;">
{{ getCuentaPeriodo(cuenta.cuentasPeriodo,periodo,true, true) }}
</p>
</td>
</tr>
<!--INVERSIONES-->
<tr *ngIf="flujoFondos.INVERSIONES as flujo" class="mat-row">
<td class="mat-cell ">
<p class="flujo-column">
{{ flujo.descripcion }}
</p>
<p *ngFor="let cuenta of flujo.cuentas;">
{{ cuenta.descripcion }}
</p>
</td>
<td *ngFor="let periodo of periodos;" class="mat-cell value-column">
<p>
</p>
<p *ngFor="let cuenta of flujo.cuentas;">
{{ getCuentaPeriodo(cuenta.cuentasPeriodo,periodo,true, true) }}
</p>
</td>
</tr>
<!--FLUJO_DE_FONDOS-->
<tr *ngIf="flujoFondos.FLUJO_DE_FONDOS as flujo" class="mat-row">
<td class="mat-cell ">
<p class="flujo-column">
{{ flujo.descripcion }}
</p>
</td>
<td *ngFor="let periodo of periodos;" class="mat-cell value-column flujo-column">
{{ getCuentaPeriodo(flujo.montosPeriodo,periodo,true, false) }}
</td>
</tr>
</table><file_sep>/src/app/escenarios.service.spec.ts
import { TestBed } from '@angular/core/testing';
import { EscenariosService } from './escenarios.service';
describe('EscenariosService', () => {
beforeEach(() => TestBed.configureTestingModule({}));
it('should be created', () => {
const service: EscenariosService = TestBed.get(EscenariosService);
expect(service).toBeTruthy();
});
});
<file_sep>/src/app/cursos.service.ts
import { Injectable } from '@angular/core';
import { HttpClient, HttpErrorResponse } from '@angular/common/http';
import { MessagesService } from './messages.service';
import { environment } from '../environments/environment';
import { catchError, map } from 'rxjs/operators';
@Injectable({
providedIn: 'root'
})
export class CursosService {
constructor(private http: HttpClient, private messageService: MessagesService) { }
getCursos() {
return this.http.get(`${environment.proyectoServiceHost}/api/curso`)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
getCurso(cursoId) {
return this.http.get(`${environment.proyectoServiceHost}/api/curso`)
.pipe(
map((cursos: Array<any>) => cursos.find(c => c.id === cursoId)),
catchError(this.messageService.catchError.bind(this.messageService)));
}
removeCurso(curso) {
return this.http.delete(`${environment.proyectoServiceHost}/api/curso/${curso.id}`)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
createCurso(curso) {
return this.http.post(`${environment.proyectoServiceHost}/api/curso`, curso)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
modifyCurso(curso) {
return this.http.put(`${environment.proyectoServiceHost}/api/curso/${curso.id}`, curso)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
}
<file_sep>/src/app/proyecto.service.ts
import { Injectable, Component } from '@angular/core';
import { environment } from '../environments/environment';
import { HttpClient, HttpErrorResponse } from '@angular/common/http';
import { Observable, of } from 'rxjs';
import { map, catchError } from 'rxjs/operators';
import { MessagesService } from './messages.service';
import { descripcionesTipoCuentas, descripcionesTipoFlujoFondos } from './valores-dominio';
@Injectable()
export class ProyectoService {
constructor(private http: HttpClient,
private messageService: MessagesService) { }
getEstado(idProyecto, forecast): Observable<any> {
return this.http.get(`${environment.proyectoServiceHost}/api/proyecto/${idProyecto}/estado/actual${forecast ? '-forecast' : ''}`)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
getEstados(proyectoId, forecast): Observable<any> {
return this.http.get(`${environment.proyectoServiceHost}/api/proyecto/${proyectoId}/estados${forecast ? '-forecast' : ''}`)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
forecast(idProyecto, forecast) {
return this.http.post(`${environment.proyectoServiceHost}/api/proyecto/${idProyecto}/forecast`, forecast)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
modalidadCobro(idProyecto, modalidadCobro) {
return this.http.post(`${environment.proyectoServiceHost}/api/proyecto/${idProyecto}/modalidadCobro`, modalidadCobro)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
proveedorSeleccionado(idProyecto, proveedorSeleccionado) {
return this.http.post(`${environment.proyectoServiceHost}/api/proyecto/${idProyecto}/proveedor`, proveedorSeleccionado)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
simular(idProyecto, opciones) {
return this.http.post(`${environment.proyectoServiceHost}/api/proyecto/${idProyecto}/simular-forecast`, opciones)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
getFlujoFondos(idProyecto, forecast): Observable<any> {
return this.http.get(`${environment.proyectoServiceHost}/api/proyecto/${idProyecto}/flujo-fondo${forecast ? '-forecast' : ''}`)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
getPresupuestoFinanciero(idProyecto,forecast): Observable<any> {
return this.http.get(`${environment.proyectoServiceHost}/api/proyecto/${idProyecto}/presupuesto-financiero${forecast ? '-forecast' : ''}`)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
getPresupuestoEconomico(idProyecto,forecast): Observable<any> {
return this.http.get(`${environment.proyectoServiceHost}/api/proyecto/${idProyecto}/presupuesto-economico${forecast ? '-forecast' : ''}`)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
getPeriodoActual(idProyecto, forecast) {
return this.getEstado(idProyecto, forecast).pipe(map(estado => estado.proyecto.escenario.maximosPeriodos))
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
getDecisiones(idProyecto): Observable<any> {
return this.http.get(`${environment.proyectoServiceHost}/api/proyecto/${idProyecto}/decisiones`)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
getForecast(idProyecto) {
return this.http.get(`${environment.proyectoServiceHost}/api/proyecto/${idProyecto}/forecast`)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
getModalidadCobro(idProyecto) {
return this.http.get(`${environment.proyectoServiceHost}/api/proyecto/${idProyecto}/modalidadCobro`)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
getProveedores(idProyecto): Observable<any> {
return this.http.get(`${environment.proyectoServiceHost}/api/proyecto/${idProyecto}/proveedor`)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
getTipoCuentas() {
return this.http.get(`${environment.proyectoServiceHost}/api/tipoCuentas`)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)),
map(tipoCuentas => tipoCuentas.map(tipoCuenta => {
return {
key: tipoCuenta,
descripcion: descripcionesTipoCuentas[tipoCuenta]
}
})));
}
getTipoFlujoFondos() {
return this.http.get(`${environment.proyectoServiceHost}/api/tipoFlujoFondos`)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)),
map(tipoFlujoFondos => tipoFlujoFondos.map(tipoFlujoFondo => {
return {
key: tipoFlujoFondo,
descripcion: descripcionesTipoFlujoFondos[tipoFlujoFondo]
}
})));
}
getBalanceFinal(idProyecto,forecast) {
return this.http.get(`${environment.proyectoServiceHost}/api/proyecto/${idProyecto}/balance-final${forecast ? '-forecast' : ''}`)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
getCredito(idProyecto): Observable<any> {
return this.http.get(`${environment.proyectoServiceHost}/api/proyecto/${idProyecto}/financiacionTomado`)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
tomarCredito(credito) {
return this.http.post(`${environment.proyectoServiceHost}/api/proyecto/${credito.proyectoId}/credito`, credito)
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
entregarProyecto(idProyecto) {
return this.http.post(`${environment.proyectoServiceHost}/api/proyecto/${idProyecto}/entregar`, {})
.pipe(catchError(this.messageService.catchError.bind(this.messageService)));
}
}
<file_sep>/src/app/financiacion.service.spec.ts
import { TestBed } from '@angular/core/testing';
import { FinanciacionService } from './financiacion.service';
describe('FinanciacionService', () => {
beforeEach(() => TestBed.configureTestingModule({}));
it('should be created', () => {
const service: FinanciacionService = TestBed.get(FinanciacionService);
expect(service).toBeTruthy();
});
});
<file_sep>/src/app/resultados-admin/resultados-admin.component.ts
import { Component, OnInit, Inject } from '@angular/core';
import { EscenariosService } from '../escenarios.service';
import { ActivatedRoute, Router } from '@angular/router';
import { UsuarioService } from '../usuario.service';
import { ProyectoService } from '../proyecto.service';
import { MatDialogRef, MAT_DIALOG_DATA, MatDialog } from '@angular/material';
@Component({
selector: 'app-resultados',
templateUrl: './resultados-admin.component.html',
styleUrls: ['./resultados-admin.component.css']
})
export class ResultadosAdminComponent implements OnInit {
proyecto;
escenario;
decisiones;
escenarioCurso;
forecast;
seccion = "RESULTADOS_FORECAST";
cursoId;
usuarioId;
constructor(private escenarioService: EscenariosService, private route: ActivatedRoute,
private usuarioService: UsuarioService, private proyectoService: ProyectoService, public dialog: MatDialog, private router: Router) { }
ngOnInit() {
var escenarioId = Number(this.route.snapshot.paramMap.get('escenarioId'));
this.cursoId = Number(this.route.snapshot.paramMap.get('cursoId'));
this.usuarioId = Number(this.route.snapshot.paramMap.get('usuarioId'));
this.getEscenario(escenarioId);
this.getProyecto(escenarioId, this.usuarioId);
this.getDetalleEscenarioUsuariosPorCurso(escenarioId, this.cursoId);
}
getDetalleEscenarioUsuariosPorCurso(escenarioId, cursoId) {
this.escenarioService.getDetalleEscenarioUsuariosPorCurso(escenarioId, cursoId).subscribe(escenarioCurso => {
this.escenarioCurso = escenarioCurso;
})
}
getProyecto(escenarioId, usuarioId) {
this.usuarioService.getProyecto(escenarioId, usuarioId).subscribe(estado => {
this.proyecto = estado.proyecto;
this.getDecisiones(this.proyecto.id);
this.getForecast(this.proyecto.id);
})
}
getDecisiones(proyectoId) {
this.proyectoService.getDecisiones(proyectoId)
.subscribe(decisiones => this.decisiones = decisiones);
}
getEscenario(escenarioId) {
this.escenarioService.getEscenario(escenarioId).subscribe(escenario => this.escenario = escenario);
}
getForecast(proyectoId) {
this.proyectoService.getForecast(proyectoId).subscribe(forecast => {this.forecast = forecast;
if(forecast.length <= 0) this.seccion = "RESULTADOS_REALES"});
}
}
<file_sep>/src/app/home/home.component.ts
import { Component, OnInit, ViewChild } from '@angular/core';
import { MatSidenav, MatDialog } from '@angular/material';
import { Router } from '@angular/router';
import { AngularFireAuth } from '@angular/fire/auth';
import { UsuarioService } from '../usuario.service';
import { MatriculacionDialogComponent } from '../matriculacion-dialog/matriculacion-dialog.component';
import { MediaMatcher } from '@angular/cdk/layout';
@Component({
selector: 'app-home',
templateUrl: './home.component.html',
styleUrls: ['./home.component.css']
})
export class HomeComponent implements OnInit {
@ViewChild('sidenav', {
static: true
}) sidenav: MatSidenav;
user: any;
year = new Date().getFullYear();
usuario;
opened = true;
pendingRequests;
mobileQuery: MediaQueryList;
constructor(private router: Router,
private afAuth: AngularFireAuth,
private usuarioService: UsuarioService,
private dialog: MatDialog,
private media: MediaMatcher) {
this.mobileQuery = this.media.matchMedia('(max-width: 600px)');
}
ngOnInit() {
this.afAuth.authState.subscribe(user => {
if (user && !this.user) {
this.user = user;
this.getUsuario({
mail: user.email,
nombreCompleto: user.displayName || user.email,
fotoUrl: user.photoURL
});
} else {
// User is not logged in
this.router.navigateByUrl("/login");
}
});
}
getUsuario(mail) {
this.usuarioService.getUsuario(mail).subscribe(usuario => {
this.usuario = usuario
if (!usuario.curso && usuario.rol === "JUGADOR") {
this.matricularUsuario(usuario);
}
}
)
}
matricularUsuario(usuario) {
const dialogRef = this.dialog.open(MatriculacionDialogComponent, {
width: '400px',
data: usuario,
disableClose: true
});
dialogRef.afterClosed().subscribe(_ => {
this.router.navigateByUrl('/simulaciones', { skipLocationChange: true })
this.getUsuario(usuario)
});
}
close() {
this.sidenav.close();
}
logout() {
this.afAuth.auth.signOut();
}
}
| 077aef0a2a72045b231e71867ca699b2fc0602e4 | [
"Markdown",
"TypeScript",
"HTML"
] | 51 | TypeScript | pf2019g4/utn-simulador-ui | 3e8b3b23cf17c45a57bab40a89694421fdc5c100 | 466c6c63df5bfc4e15d062b54b084695bbc24373 | |
refs/heads/master | <file_sep>Test::Unit::TestCase.fixture_path = File.expand_path(File.dirname(__FILE__)) + "/fixtures/"
$LOAD_PATH.unshift(Test::Unit::TestCase.fixture_path)
Fixtures.create_fixtures(Test::Unit::TestCase.fixture_path, %w(countries languages translations).collect{|table_name| "globalize_#{table_name}"})
class Test::Unit::TestCase
def uses_config_file(config_file, &block)
old_config_file = Locale.config_file
Locale.config_file = old_config_file.gsub(/\w+\.\w+$/, config_file)
Locale.configuration = false
yield
Locale.configuration = false
Locale.config_file = old_config_file
end
end
module Globalize #:nodoc:
class Locale #:nodoc:
cattr_writer :formatting, :configuration
end
module Helpers #:nodoc:
def self.click_partial #:nodoc:
@@click_partial
end
end
end
| 34a740e4efe651b5eabca84d3666ee8753a29832 | [
"Ruby"
] | 1 | Ruby | HaFrancisca/click-to-globalize | fda5c0d121f90e48dbbe2380bfdfb66ede847117 | bb5176c0a0a9d8760a1546692d92170b7f27468f | |
refs/heads/master | <file_sep>import pyautogui as pg
import time
pg.hotkey ("winleft","ctrl","d")
pg.hotkey ("winleft")
pg.typewrite ("chrome\n",.1)
pg.hotkey ("winleft", "up")
pg.typewrite ("netflix.com\n", .5)
time.sleep(2)
pg.hotkey ('tab')
pg.hotkey ('tab')
pg.hotkey ("enter")
time.sleep (1)
pg.hotkey ("tab")
pg.typewrite ("<EMAIL>",.2)
pg.hotkey ('tab')
pw=pg.password('Enter password here','<PASSWORD>','','*')
pg.typewrite(pw+"\n", 0.3)
pw = ""
<file_sep>import webbrowser
import pyautogui as pg
fails = ["https://www.youtube.com/watch?v=IqTk1je6iYs"]
vines = ["https://www.youtube.com/watch?v=x0uXVy4fzUA"]
answer = pg.prompt(
"""
Which would you like to do?
a) Watch fails
b) Watch best vines of 2017
"""
)
if answer == "a":
for i in videos:
webrowser.open(i)
elif answer == "b":
for i in music:
webbrowser.open(i)
<file_sep>import win32com.client as wincl
speak = wincl.Dispatch("SAPI.SpVoice")
speak.Speak("<NAME> in the chat.")
answer = pg.prompt("Enter your favorite color.")
if answer == "orange":
speak.Speak("My favorite color is orange too! We must be twins.")
elif answer == "blue":
speak.Speak("I don't like blue as much as IO like orange.")
else:
speak.Speak("You liker the color " + answer)
speak.Speak("What kind of video should we watch?")
video = pg.prompt("Enter video below.")
speak.Speak("Ok, let's look for" + video + "on youtube and see what we find.")
wb.open("https://www.youtube.com/results?search_query=" + video)
<file_sep>name = "Lilja"
state = "hawaii"
city = "New York"
tvshow = "manhunt"
book = "Birlin Boxing Club"
print("Hello " + name)
print("have you visited " + state + " or " + city + " recently?")
print("Yes, I visited Hawaii!")
print("where did you go in " + state + "?" )
print("What is your favorite class?")
subject = input()
if subject == "History"
print("Me too!")
else:
print(subject + " is a great class!"
if subject == "Math"
else:
print ("Ew I hate Math")
<file_sep># pyautogui
Automation
<file_sep>import pyautogui as pg
pg.moveTo(1293, 10,3)
pg.click(1293, 10, 1)
<file_sep>name = "<NAME>"
subjects = ["English","spanish","Math","History","Science"]
print ("My name is " + name)
#print(subjects)
for i in subjects:
print("One of my classes is " + i)
colors = ["Pink","Blue","Purple","Green","Red"]
for i in colors:
if i == "blue":
print (i + " is the best color ever1")
elif i == "Red":
print (i + " is the worst color ever")
elif i == "Green":
print (i + " is the worst color ever")
elif i == "Purple":
print (i + " is the worst color ever")
elif i == "Pink":
print (i + " is the worst color ever")
print("The best color is " + i)
colors = []
while True:
print("wHAT are your favorite colors? Type 'end' to quit.")
answer = input()
if answer == "end":
break
else:
colors.append(answer)
for i in colors:
print ("One of you favorite colors is " + i)
<file_sep>import pyautogui as pg
import time
import webbrowser
points = 0
#question
answer =pg.prompt(
"""
Which would you rather do?
a) slap battle with a friend
b) get a butterfly tatoo
"""
)
# Give points
if answer == "a":
points +=1
elif answer == "b":
points += 2
# END OF SURVEY
pg.alert("You are.....")
#ted
if points == 2:
pg.alert ("Ted")
webrowser.open("https://uproxx.files.wordpress.com/2014/09/ted-mosby.jpg?quality=100&w=650")
#barney
elif points == 1:
pg.alert ("Barney")
webbrowser.open ("https://i.imgur.com/aDCdAyb.gif")
<file_sep>import time
### MAD LIBS ###
### scource: https://www.google.com/search?sa=G&hl=en&q=mad+libs+printable+free&tbm=isch&tbs=simg:CAQSlwEJ5j-b1v4OVX4aiwELEKjU2AQaBAgUCAoMCxCwjKcIGmIKYAgDEii-Hb0dwR2QE4oTwB2_1HYsTkRP1B8o_15D3LP-U93TTmPec92zTJNt40GjCLuiy9t-1BW6cRRWjyAw1lbwRb_1C-73ijzlI17lkzOxASCb2ocBJYZ5V4z0_1TU3mQgBAwLEI6u_1ggaCgoICAESBJRzf8EM&ved=0ahUKEwim39fBiofXAhXM4IMKHZQuDXgQwg4IIygA&biw=763&bih=351&dpr=1.75#imgrc=X32CJ6QabHwUPM: ###
### Ask Questions here ###
print("write a adjective")
adj1 = input()
print("write a noun")
noun1 = input()
print("Write an adjective")
adj2 = input()
print("Write a plural noun")
plnoun1 = input()
print("Write an adverb")
adverb1 = input()
print("Write a verb ending in ING")
verbING1 = input()
print("Write a silly word plural")
sillyplural = input()
print("Write a adjective")
adj3 = input()
print("Write a plural noun")
plnoun2 = input()
print("Write a First name")
fn1 = input()
print("Write an adjective")
adj4 = input()
print("Write a number")
number1 = input()
print("Write another first name")
fn2 = input()
print("Write another first name")
fn3 = input()
print("Write another first name")
fn4 = input()
print("Write another first name")
fn5 = input()
print("Write another first name")
fn6 = input()
print("Write another first name")
fn7 = input()
print("Write a Plural Noun")
plnoun3 = input()
### tell the MadLib ###
print("When we lookj up into the sky on a/an " + adj1 + " summer night, we see millions of tiny spots of light.")
print("Each one represents a/an " + noun1 + "which is the center of a/an" + adj2 + " solar system with dozens of " + plnoun1 + " revolving " + adverb1 + " around a distant sun ")
print("Somtimes these suns expand and begin " + verbING1 + " their neighbors.")
print("Soon they will become so big, they will turn into " + sillyplural)
print("Eventually they subside and become " + adj3 + "giants or perhaps black " + plnoun2)
print("Our own planet, which we cal l" + fn1 + "circles around our " + adj4 + "sun " + number1 + " times every year")
print("There are eight other planets in out solar system.")
print("They are named " + fn2 + " " + fn3 + " " + fn4 + " " + fn5 + " " + fn6 + " " + fn7 + " Jupiter, and Mars.")
print("Scientists who study these planets are called " + plnoun3 + " .")
time.sleep (130)
<file_sep>import random
words = ["apple","computer","pen","girl","sticker"]
hint1 = ["fruit","technology","writing utensel","Gender","sticky"]
hint2 = ["Comes from tree","key board","uses ink","pink","often used to decorate computers"]
number = random.randint(0,4)
secretword = words[number]
guess = ""
counter = 0
while True:
print("Guess the secret word!")
print("Type 'hint1', 'hint2', 'first letter', 'last letter', or 'give up' for help.")
guess = input()
if guess ==secretword:
print("you win!")
break
elif guess == "hint1":
print( hint1[number] )
elif guess == "hint2":
print( hint2[number] )
elif guess == "first letter":
print( secretword[-1] )
elif guess == "last letter1":
print( secretword[-1] )
elif guess == "give up":
print("The last word was" + secretword )
break
else:
counter +=1
<file_sep>print("what is your favorite Movie?")
show = input ()
if show == "Mean Girls":
print("Who is your favorite character?")
character = input()
if character == "<NAME>":
print ("She is my favorite character too!")
print("What is your favorite book?")
book = input()
if "wonder" in book:
print("I like the book, but they are comming out with a movie!")
print("What is your favorite color?")
color = input()
if "blue" in color:
print("My favorite color is blue too!")
if "pink" in color:
print("Pink is a really cool color!")
| a3cd868c30164cd815ad61fad154bb6a573d512c | [
"Markdown",
"Python"
] | 11 | Python | LiljaK/pyautogui | 62b82582aad8ea833e4577277074de50b972ca4b | 6233b1141707862a448c507dd551b5309c5e1c31 | |
refs/heads/master | <repo_name>xiaochuan1719/vue-learning<file_sep>/simple-chapter/chapter01/index.js
// 1. 创建一个 Vue 对象
const vm = new Vue({
el: '#main',
data: {
message: 'Hello, Vue!'
}
});
vm.message = 'Hello, World !!';<file_sep>/simple-chapter/chapter04/index01.js
const vm = new Vue({
el: '#main',
data: {
redText: 'text-red',
isRed: true,
isBold: false,
classObj: {
'text-red': true,
'text-bold': false
},
isTextRed: true,
textRedClass: 'text-red',
textBoldClass: 'text-bold'
},
methods: {
update01() {
this.redText = 'text-green';
},
update02() {
this.isRed = false;
this.isBold = true;
},
update03() {
this.isTextRed = false;
}
},
computed: {
classObject: function () {
return {
'text-red': this.isRed && this.isBold,
'text-bold': this.isRed && !this.isBold
}
}
}
});<file_sep>/simple-chapter/chapter08/index03.js
const vm = new Vue({
el: '#main',
data: {
msg: '',
age: '',
msgText: ''
}
});
<file_sep>/simple-chapter/chapter06/index02.js
const vm = new Vue({
el: '#main',
data: {
exampleObj: {
name: 'vue.js',
desc: 'The Progressive JavaScript Framework',
version: '2.5.17',
author: '尤雨溪'
}
}
});<file_sep>/simple-chapter/chapter08/index02.js
const vm = new Vue({
el: '#main',
data: {
toggle: '',
pick: '',
aa: 'aa',
selected: ''
}
});<file_sep>/README.md
## vue-learning
Learn Vue2: Step By Step
<file_sep>/simple-chapter/chapter06/todo-list-example.js
/**
* TodoList item 组件
* 任务列表组件
*/
Vue.component('todo-item', {
template: `
<li>
{{ title }}
<a href="#" v-on:click="$emit('remove')"> X</a>
</li>
`,
props: ['title']
});
const vm = new Vue({
el: '#todo-list-example',
data: {
newTodoText: '',
todos: [
{id: 1, title: 'Feed the Cats'},
{id: 2, title: 'Read a book'},
{id: 3, title: 'Thinking my lover'}
],
nextTodoId: 4
},
methods: {
addNewTodo: function () {
this.todos.push({
id: this.nextTodoId++,
title: this.newTodoText
});
this.newTodoText = '';
}
}
});<file_sep>/simple-chapter/chapter04/index02.js
/**
* - CSS 属性名可以用驼峰式 (camelCase) 或短横线分隔 (kebab-case,记得用单引号括起来) 来命名
* - 直接绑定到一个样式对象通常更好,使得模板简洁清晰;该对象属性及CSS属性名,可以使用驼峰式和kebab-case式
* - 对象语法常常结合返回对象的计算属性使用
*/
const vm = new Vue({
el: '#main',
data: {
bgColor: 'green',
textColor: 'red',
fontSize: 20,
styleObject: {
backgroundColor: 'yellow',
color: '#000',
fontSize: '30px'
}
}
});
<file_sep>/simple-chapter/chapter09/readme.md
## Components Baseic
```js
Vue.component('button-counter', {
data: function () {
return {
count: 0
}
},
template: '<button v-on:click="count++">You clicked me {{ count }} times.</button>'
});
const vm = new Vue({
el: '#main'
});
```
```html
<div id="component-example-01">
<button-counter></button-counter>
</div>
```
#### #data 必须是一个函数
```vuejs
// data 选项必须是一个函数
Vue.component('button-counter', {
data: function () {
return {
count: 0
}
},
template: '<button v-on:click="count++">You clicked me {{ count }} times.</button>'
});
```
#### #通过Prop向子组件传递数据
```vuejs
Vue.component('button-counter', {
props: ['title'],
template: '<button v-on:click="count++">You clicked {{ title }} {{ count }} times.</button>'
});
```
#### #每个组件必须只有一个根元素
#### #通过事件向父级组件发送消息
<file_sep>/simple-chapter/chapter02/readme.md
## Template Syntax
### Interpolations 插值
#### Text 文本
- Mustache 语法
数据绑定最常见的形式就是使用“Mustache”语法 (双大括号) 的文本插值:
```
<p>Message: {{ message }}</p>
<p>Message: {{ message.toUpperCase() }}</p>
```
- Vue 指令
**v-text 和 v-html**
```
<p v-html="message"></p>
<p v-text="data"></p>
// vue 实例
const vm = new Vue({
el: '#main',
data: {
message: '<a href="https://vuejs.org/">https://vuejs.org/</a>'
}
});
```
**v-cloak 和 v-text指令**
v-cloak 独立指令,不需要表达式。这个指令保持在元素上直到关联实例结束编译。
常和 CSS 规则: `[v-cloak]{display: none;}` 一起使用,可以隐藏未编译的 Mustache 标签直到实例结束编译。可以解决使用 Mustache 标签插值时的闪烁问题。(配合CSS规则,可以实现很多的效果哦!!)
```css
[v-cloak] {
display: none;
}
```
```html
<span v-cloak>{{ message }}</span>
```
v-text 需要表达式,默认是没有插值闪烁问题的,且会覆盖元素里面所有的内容;而使用插值表达式,则只会覆盖表达式部分的内容。
**v-bind 指令**
```
<!-- v-bind 指令 -->
<p>Vue logo: <img v-bind:src="logo_imgUrl" alt="" width="50px" height="50px"></p>
<p>Vue logo: <img :src="logo_imgUrl" alt="" width="50px" height="50px"></p>
```
**v-on 指令**
```
<!-- v-on 指令 -->
<a href="#" v-on:click="alertGo">Link one</a>
<br>
<a href="#" @click="confirmGo(tips)">Link two</a>
```
<file_sep>/simple-chapter/chapter04/readme.md
## Class and Style Bindings
class 和 style 绑定就是专门用来实现动态样式效果的技术
### Binding HTML Classes 绑定HTML class
### #String Syntax
- v-bind:class='data属性'
- data属性的属性值为类名
### #Object Syntax
We can pass an object to v-bind:class to dynamically toggle classes:
```html
<div v-bind:class="{ active: isActive }"></div>
```
- v-bind:class=‘{xxx: boolVal, xxx: boolVal}';
- 类名 xxx 的存在与否取决于数据属性 boolVal 的 Truthy;
- 绑定的数据对象不必内联定义在模板里,如下所示:
```html
<div v-bind:class="classObject"></div>
```
```js
new Vue({
data: {
classObject: {
active: true,
'text-danger': false
}
}
});
```
- 绑定一个返回对象的计算属性;这是一个常用且强大的模式:
```html
<div v-bind:class="classObject"></div>
```
```js
new Vue({
data: {
isRed: true,
isBold: false
},
computed: {
classObject: function () {
return {
'text-red': this.isRed && this.isBold,
'text-bold': this.isRed && !this.isBold
}
}
}
});
```
### #Array Syntax
We can pass an array to v-bind:class to apply a list of classes:
```html
<div v-bind:class="[activeClass, errorClass]"></div>
```
- v-bind:class="[xxxx, xxxx]";
- xxxx 为data选项属性,该属性值为 类名
- 根据条件切换列表中的 class,可以用三元表达式
```html
<div v-bind:class="[isActive ? activeClass : '', errorClass]"></div>
```
- 当有多个条件 class 时,上述写法有些繁琐。所以在数组语法中也可以使用对象语法
```html
<div v-bind:class="[{ active: isActive }, errorClass]"></div>
```
### Binding Inline Styles 绑定内联样式
### #Object Syntax
```html
<div :style="{ color: textColor, fontSize: fontSize + 'px' }"></div>
<div :style="styleObj"></div>
```
```js
new Vue({
el: '',
data: {
textColor: 'red',
fontSize: 20,
styleObj: {
textColor: 'green',
fontSize: '30px'
}
}
});
```
- CSS 属性名可以用驼峰式 (camelCase) 或短横线分隔 (kebab-case,记得用单引号括起来) 来命名
- 直接绑定到一个样式对象通常更好,使得模板简洁清晰;该对象属性及CSS属性名,可以使用驼峰式和kebab-case式
- 对象语法常常结合返回对象的计算属性使用
### #Array Syntax
v-bind:style 的数组语法可以将多个样式对象应用到同一个元素上
```html
<div :style="[baseStyles, overridingStyles]"></div>
```
<file_sep>/simple-chapter/chapter03/readme.md
## Computed Properties and Watchers
### Computed Properties 计算属性
模板里面使用表达式非常方便,但尤大大最初设计的初衷只是做一些简单的运算。如果模板中放入过多的逻辑,将会难以阅读和维护,如:
```vue
<div id="example">
{{ message.split('').reverse().join('') }}
</div>
```
所以,对于任何复杂的逻辑,都应当选择使用**计算属性**
### #举个栗子
```html
<div id="main">
<P>Original message: "{{ message }}"</P>
<p>Reverse message by computed: "{{ reverseMessage }}"</p>
<p>Reverse message by methods: "{{ reverseWords() }}"</p>
</div>
```
```js
const vm = new Vue({
el: '#main',
data: {
message: 'Hello'
},
// 这里声明了一个 reverseMessage 计算属性,提供的函数作为该属性的 getter 函数
computed: {
// a computed getter
reverseMessage: function () {
// 'this' points to the vm instance
return this.message.split('').reverse().join('');
},
currentDate1: function () {
return Date.now();
}
},
// 这里声明了一个 reverseWords 的方法
methods: {
reverseWords: function () {
return this.message.split('').reverse().join('');
},
currentDate2: function () {
return Date.now();
}
},
// 监听器
watch: {
// 监听 data 的 firstName 属性是否发生了变化,如果发生变化,则更新
firstName: function (val) {
this.fullName = val + ' ' + this.lastName;
}
}
});
// 除了使用 watch 选项来实现监听,vue 还提供了命令式 API,如:vm.$watch();
vm.$watch('lastName', function (val) {
this.fullName = this.firstName + ' ' + val;
});
```
### Computed Properties and Methods 计算属性与方法
- 计算属性的执行时机:初始化时 / 相关属性发生改变时
- **计算属性是基于它们的依赖(相关属性)进行缓存的**
1. 在控制台执行 vm.currentDate1 ,发现还是原来的值
2. 在控制台执行 vm.currentDate2 ,发现值改变了
>我们为什么需要缓存?假设我们有一个性能开销比较大的计算属性 A,它需要遍历一个巨大的数组并做大量的计算。然后我们可能有其他的计算属性依赖于 A 。
如果没有缓存,我们将不可避免的多次执行 A 的 getter!如果你不希望有缓存,请用方法来替代。
### Computed Properties and Watcher 计算属性与监听器
Vue does provide a more generic way to observe and react to data changes on a Vue instance: watch properties.
### Computed Setter 计算属性的setter
Computed properties are by default getter-only, but you can also provide a setter when you need it:
```js
const vm = new Vue({
el: '#main',
data: {
firstName: '',
lastName: ''
},
computed: {
fullName: {
// 当需要读取当前属性时回调,根据相关属性计算并返回当前属性的值
get() {
return this.firstName + ' ' + this.lastName;
},
// 监视当前属性值的变化,当属性值发生改变时回调,更新相关的属性数据
set(value) {
const names = value.split(' ');
this.firstName = names[0];
this.lastName = names[1];
}
}
}
});
```
### Watchers
While computed properties are more appropriate in most cases, there are times when a custom watcher is necessary.
That’s why Vue provides a more generic way to react to data changes through the watch option. This is most useful
when you want to perform asynchronous or expensive operations in response to changing data.
>当需要在数据变化时执行异步或开销较大的操作时,这个方式是最有用的。
<file_sep>/simple-chapter/chapter05/index01.js
/**
*
*/
const vm = new Vue({
el: '#main',
data: {
isView: true,
isViewTemplate: true,
type: 'B'
}
});<file_sep>/simple-chapter/chapter07/index01.js
const vm = new Vue({
el: '#main',
data: {
counter: 0
},
methods: {
addCounter: function () {
this.counter += 2;
},
showEventButton: function (event) {
alert('the counter = ' + this.counter);
if (event) {
alert('this tag name: ' + event.target.tagName); // BUTTON
}
},
say: function (message, event) {
alert(message);
if (event) {
alert('this tag name: ' + event.target.tagName);
}
}
}
});<file_sep>/simple-chapter/chapter05/readme.md
## Conditional Rendering
### v-if
根据表达式的值的真假条件渲染元素。
在切换时元素及它的数据绑定/组件被销毁并重建。如果元素是 <template> ,将提出它的内容作为条件块。
当条件变化时该指令触发过渡效果。
当 v-for 与 v-if 一起使用时,v-for 的优先级高于 v-if 。
### 用**key**管理可复用的元素
Vue会尽可能高效地渲染元素,通常会复用已有元素而不是从头开始渲染。给相同的元素都添加一个具有唯一值的 `key` 属性即可:
```html
<template v-if="loginType === 'username'">
<label>UserName</label>
<input type="text" placeholder="Enter your username" key="username-input">
</template>
<template v-else>
<label>Email</label>
<input type="text" placeholder="Enter your email addr" key="email-input">
</template>
<button @click="toggleLoginType">Toggle login type</button>
```
```js
const vm = new Vue({
el: '#main',
data: {
loginType: 'username'
},
methods: {
toggleLoginType () {
this.loginType === 'username' ? this.loginType = 'email' : this.loginType = 'username';
}
}
});
```
如上代码所示,如果不添加 `key` 属性,则两个 input 元素为了更高效地渲染,会进行复用;如果添加了 `key` ,两个 input 元素将完全独立,不再复用。
### v-show 指令
```html
<template v-show="isShow">
<p>根据条件展示元素的选项</p>
<p>不同的是带有 v-show 的元素始终会被渲染并保留在 DOM 中。v-show 只是简单地切换元素的 CSS 属性 display。</p>
</template>
<button @click="toggleShow">Toggle Show</button>
```
```js
const vm = new Vue({
el: '#main',
data: {
isShow: false
},
methods: {
toggleShow () {
this.isShow = !this.isShow;
}
}
});
```
点击按钮,页面内容并没有发生变化,这是由于 `v-show` 指令不支持 `<template>` 元素,且 `v-else` 也不支持 `<template>` 元素。
```html
<div class="content" v-show="isShow">
<p>根据条件展示元素的选项</p>
<p>不同的是带有 v-show 的元素始终会被渲染并保留在 DOM 中。v-show 只是简单地切换元素的 CSS 属性 display。</p>
</div>
<button @click="toggleShow">Toggle Show</button>
```
点击按钮,页面内容会隐藏显示交互变化。
> **v-if 和 v-show 的区别**
`v-if` 是“真正”的条件渲染,因为它会确保在切换过程中条件块内的事件监听器和子组件适当地被销毁和重建。
`v-show` 简单地切换元素的 CSS 属性 display ;不管初始条件是什么,元素总是会被渲染
<file_sep>/simple-chapter/chapter06/index01.js
const vm = new Vue({
el: '#main',
data: {
jsFrameworkArr: [
{ name: 'Angular' },
{ name: 'Vue' },
{ name: 'React' },
{ name: 'Preact' },
{ name: 'Inferno'},
{ name: 'Nerv' },
{ name: 'dva' }
],
javaFrameworkArr: [
{ name: 'Spring' },
{ name: 'SpringBoot' },
{ name: 'SpringCloud' },
{ name: 'SpringData' }
]
}
});
<file_sep>/simple-chapter/chapter06/readme.md
## List Rendering
### Mapping an Array to Elements with `v-for`
```html
<h3>1. 用 v-for 把一个数组对应为一组元素</h3>
<ul id="example01">
<h4>JavaScript Framework</h4>
<li v-for="item in jsFrameworkArr">
{{ item.framework_name }}
</li>
</ul>
```
```js
const vm = new Vue({
el: '#main',
data: {
jsFrameworkArr: [
{ framework_name: 'Angular' },
{ framework_name: 'Vue' },
{ framework_name: 'React' },
{ framework_name: 'Preact' },
{ framework_name: 'Inferno'},
{ framework_name: 'Nerv' },
{ framework_name: 'dva' }
]
}
});
```
> 在 `v-for` 块中,我们拥有对父作用域属性的完全访问权限;
> 除了使用 `item in items` 形式语法外,也支持使用 `item of items` 形式的语法;
> `v-for` 还支持一个可选的第二个参数为当前项的索引;
```html
<h4>Java Framework</h4>
<ul id="example02">
<li v-for="(item, index) of javaFrameworkArr">
{{ index + 1 }} > {{ item.name }}
</li>
</ul>
```
```js
const vm = new Vue({
el: '#main',
data: {
javaFrameworkArr: [
{ name: 'Spring' },
{ name: 'SpringBoot' },
{ name: 'SpringCloud' },
{ name: 'SpringData' }
]
}
});
```
### `v-for` with an Object
基本格式: v-for="(value, key, index) in object"
```html
<h3>1. v-for 迭代一个对象 </h3>
<h4>JavaScript Framework</h4>
<ul id="example01">
<li v-for="(value, key, index) in exampleObj">
{{ index }} 、 {{ key }}: {{ value }}
</li>
</ul>
```
```js
const vm = new Vue({
el: '#main',
data: {
exampleObj: {
name: 'vue.js',
desc: 'The Progressive JavaScript Framework',
version: '2.5.17',
author: '尤雨溪'
}
}
});
```
> 在遍历对象时,是按 Object.keys() 的结果遍历,但是不能保证它的结果在不同的 JavaScript 引擎下是一致的。
> 当 Vue.js 用 v-for 正在更新已渲染过的元素列表时,它默认用“就地复用”策略。
> 这个默认的模式是高效的,但是只适用于**不依赖子组件状态或临时 DOM 状态 (例如:表单输入值) 的列表渲染输出**。
### 数组更新检测
#### #编译方法
Vue 包含一组观察数组的变异方法,它们会触发视图更新。
- push()
- pop()
- shift()
- unshift()
- splice()
- sort()
- reverse()
#### #替换数组
变异方法(mutation method),会改变调用这些方法的原始数组。
非变异方法(non-mutation method)方法,如:filter(), concat(), slice() ;这些不会改变原始数组,但总会返回一个新的数组。
当使用非变异方法时,可以用新数组替换旧数组:
```js
example.items = example.items.filter(function(item) {
return item.message.match(/Foo/);
});
```
#### #注意事项
由于 JS 的限制,Vue 不能检测以下变动的数组:
1. 利用索引直接设置一个项时,如: `vm.items[indexOfItem] = newValue`
2. 修改数组长度时,如: `vm.items.length = newLength`
举个栗子:
```js
const vm = new Vue({
el: '#example'
data: {
items: ['a', 'b', 'c']
}
});
vm.items[1] = 'x';
vm.items.length = 5;
// 以上均不会响应触发视图的更新
```
解决方案:
1. 解决第一类问题
```js
// Vue.set()
Vue.set(vm.items, indexOfItem, newValue);
vm.$set(vm.items, indexOfItem, newValue);
// Array.prototype.splice
vm.items.splice(indexOfItem, 1, newValue);
```
2. 解决第二类问题
```js
vm.items.splice(newLength);
```
### 对象更改检测注意事项
**Vue 不能检测对象属性的添加或删除**
对于已经创建的 Vue 实例,Vue 不能动态添加根级别的响应式属性。但可以使用 `Vue.set(object, key, value)` 方法向嵌套对象添加响应式属性。
```js
const vm = new Vue({
data: {
userProfile: {
name: 'Anna'
}
}
});
// 嵌套对象 userProfile 中添加一个新的 age 属性
Vue.set(vm.userProfile, 'age', 20);
vm.$set(vm.userProfile, 'age', 18);
// 如果要添加多个新的响应式属性,直接通过 Object.assign(object, newObj) 是不行的,你应该这样做:
vm.userProfile = Object.assign(vm.userProfile, {
age: 18,
favoriteColor: 'red, green'
});
```
### 显示过滤/排序结果
需求:显示一个过滤或排序后的数组,而不实际改变或重置原始数据
方案:创建返回过滤或排序数组的计算属性和方法
```html
<li v-for="num in evenNumbers">{{ num }}</li>
<li v-for="num in even(numbers)">{{ num }}</li>
```
```js
const vm = new Vue({
el: '#main',
data: {
numbers: [1, 2, 3, 4, 5]
},
computed: {
evenNumbers: function() {
return this.numbers.filter(function(number) {
return number % 2 === 0;
})
}
},
methods: {
even: function(numbers) {
return numbers.filter(function(number) {
return number % 2 === 0;
});
}
}
});
```
### v-for with a Range
`v-for` can also take an integer. In this case it will repeat the template that many times.
```html
<div>
<span v-for="n in 10">{{ n }}</span>
</div>
```
### v-for on a <template>
Similar to template `v-if`, you can also use a `<template>` tag with `v-for` to render a block of multiple elements.
```html
<ul>
<template v-for="item of items">
<li>{{ item.msg }}</li>
<li class="divider" role="presentation"></li>
</template>
</ul>
```
### v-for with v-if
When they exist on the same node, `v-for` has a higher priority than `v-if` .That means the `v-if` will be run on each iteration of the loop separately.
```html
// the example only renders the todos that are not complete
<li v-for="todo in todos" v-if="!todo.isComplete">
{{ todo.msg }}
</li>
```
### v-for with a Component
You can directly use `v-for` on a custom component, like any normal element:
```html
<my-component v-for="item in items" v-bind:key="item.id"></my-component>
```
>Notice: In 2.2.0+, when using `v-for` with a component, a `key` is now required.
However, this won't automatically pass any data to the component, because components have isolated scopes of their own.
In order to pass the iterated data into the component, we should also use props:
```html
<my-component
v-for="(item, index) in itmes"
v-bind:item="item"
v-bind:index="index"
v-bind:key="item.key"
></my-component>
```
<file_sep>/simple-chapter/chapter06/index04.js
const vm = new Vue({
el: '#main',
data: {
numbers: [1, 2, 3, 4, 5]
},
computed: {
evenNumbers: function () {
return this.numbers.filter(function (number) {
return number % 2 === 0;
});
}
},
methods: {
even: function (numbers) {
return numbers.filter(function (number) {
return number % 2 === 1;
});
}
}
});
<file_sep>/simple-chapter/chapter07/readme.md
## Event Handling
### Listening to Events
`v-on` 指令可以监听 DOM 事件,并在触发时执行相关逻辑代码。
```html
<div id="example-01">
<button v-on:click="counter += 1">Add +1</button>
<p>The button above has been clicked {{ counter }} times.</p>
</div>
```
```js
const vm = new Vue({
el: '#main',
data: {
counter: 0
}
});
```
- 直接绑定一个方法
```html
<div id="example-02">
<button v-on:click="addCounter"> Add +2 </button>
<p>The button above has been clicked {{ counter }} times.</p>
</div>
```
```js
const vm = new Vue({
el: '#main',
data: {
counter: 0
},
methods: {
addCounter: function () {
this.counter += 2;
}
}
});
```
- 在内联 javascript 语句中调用方法
```html
<div id="example-03">
<button v-on:click="showEventButton">Click It</button>
<button v-on:click="say('Hi', $event)">Say Hi</button>
</div>
```
```js
const vm = new Vue({
el: '#main',
data: {
counter: 0
},
methods: {
showEventButton: function (event) {
alert('the counter = ' + this.counter);
if (event) {
alert('this tag name: ' + event.target.tagName); // BUTTON
}
},
say: function (message, event) {
alert(message);
if (event) {
alert('this tag name: ' + event.target.tagName);
}
}
}
});
```
### Event Modifiers 事件修饰符
### Key Modifiers 按键修饰符
### System Modifier Keys 系统修饰按键<file_sep>/simple-chapter/chapter01/readme.md
## Vue 实例
### 创建一个 Vue 实例
```
const vm = new Vue({
el: '#main',
data: {
message: 'Hello World'
}
});
```
### 数据与方法
### 实例生命周期钩子
### 声明周期图示
<file_sep>/simple-chapter/chapter08/readme.md
## Form Input Bindings
### Basic Usage
`v-model` 指令:在表单 `<input>`、`<textarea>`、`<select>` 元素上创建双向数据绑定。 `v-model`指令本质上是一个语法糖,负责监听用户的输入事件以更新数据。
> `v-model`会忽略所有表单元素的 `value`、`checked`、`selected` 特性的初始值,而总是将 Vue 实例的数据作为数据源,所以需要在组件的 `data` 选项中声明初始值。
#### #Text and Multiline Text
> 注意,在 `<textarea>{{mesage}}</textarea>` 文本区域中插值不会生效,应使用 `v-model` 指令代替。
Interpolation on textareas (<textarea>{{text}}</textarea>) won't work. Use v-model instead.
#### #Checkbox
Single checkbox, boolean value: (单个多选框,绑定到布尔值)
```html
<h3>3. Single Checkbox</h3>
<input type="checkbox" id="checkbox" v-model="checked">
<label for="checkbox">{{ checked }}</label>
```
Multiple checkbox, bound to the same Array: (多个复选框,绑定到同一个数组)
```html
<div id="example-04">
<h3>3. Multiple Checkbox</h3>
<input type="checkbox" id="jack" value="Jack" v-model="checkedNames">
<label for="jack">Jack</label>
<input type="checkbox" id="john" value="John" v-model="checkedNames">
<label for="john">John</label>
<input type="checkbox" id="mike" value="Mike" v-model="checkedNames">
<label for="mike">Mike</label>
<br>
<span>Checked Names: {{ checkedNames }}</span>
</div>
```
#### #Radio
#### #Select
### Value Bindings
对于单选按钮,复选框及选择框的选项,`v-model` 绑定的值通常是静态字符串(对于复选框也可以是布尔值):
```html
<!-- 当选中是,picked 为字符串 ‘a’ -->
<input type="radio" v-model="picked" value="a">
<!-- toggle 为 true 或 false -->
<input type="checkbox" v-model="toggle">
<!-- 当选中第一个选项时,selected 为字符串 ’aaa‘ -->
<select v-model="selected">
<option value=aaa">AAA</option>
</select>
```
但有时我们可能需要把值绑定到 Vue 实例的一个动态属性上,这时可以用 `v-bind` 实现,且这个属性可以不是字符串。
### Modifiers
#### #.lazy
默认情况下,`v-model` 在每次 `input` 事件触发后将输入框的值与数据进行同步
添加 `laze` 修饰符,从而转变为使用 `change` 事件进行同步(失去焦点时)
```html
<input v-model.lazy="msg" >
```
#### #.number
#### #.trim<file_sep>/simple-chapter/chapter05/index03.js
const vm = new Vue({
el: '#main',
data: {
isShow: false,
styleObj: {
color: 'red',
fontWeight: 'Bold'
}
},
methods: {
toggleShow () {
this.isShow = !this.isShow;
}
}
});<file_sep>/simple-chapter/chapter09/index01.js
Vue.component('button-counter', {
props: ['title'],
data: function () {
return {
count: 0
}
},
template: '<button v-on:click="count++">You clicked {{ title }} {{ count }} times.</button>'
});
const vm = new Vue({
el: '#main'
});
| 4aac6b8a7a5efd88df621496dc9a94e1b1147b99 | [
"JavaScript",
"Markdown"
] | 23 | JavaScript | xiaochuan1719/vue-learning | 037169656f160e079c07a1c24333cf854a27baa0 | e685ca9a6c126b5029fac6abb0771bf42f4f4e22 | |
refs/heads/master | <file_sep>import { setDefaultTimeout, runOnce } from 'bocha/node.mjs';
import { URL } from 'url';
let __dirname = new URL('.', import.meta.url).pathname;
setDefaultTimeout(3000);
runOnce(__dirname, {
fileSuffix: '.tests.js'
});<file_sep># smartdb-rediscacheprovider
A Redis cache for [smartdb](https://github.com/arnesten/smartdb). Suitable if you have many Node.js processes that
potentially makes updates to the same CouchDB documents.
## Example
```javascript
import SmartDb from 'smartdb';
import redisCacheProvider from 'smartdb-rediscacheprovider';
let db = SmartDb({
/* ... smartdb configuration ... */
cacheProvider: redisCacheProvider({ /* options */ })
});
```
## Options
The following options can be given when creating the cache provider:
* `prefix` - The key prefix to use when saving in Redis. Default is *smartdb:*
* `port` - The Redis port. Default is 6379.
* `hostname` - The Redis hostname. Default is 127.0.0.1.
* `ageThreshold` - The threshold in milliseconds for cacheMaxAge in smartdb entity settings to when Redis should be used.
If cacheMaxAge is below threshold it uses inMemoryCacheProvider instead. Default is 1000.
* `inMemoryCacheMaxAge` - The max age in milliseconds when using the inMemoryCacheProvider. Default is the value of ageThreshold.
* `inMemoryCacheMaxSize` - The max items per entity when using the inMemoryCacheProvider. Default is 1000.
## License
(The MIT License)
Copyright (c) 2013-2022 <NAME>
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in
all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
THE SOFTWARE.
| 6cbca6e29a04dab13366c8b8f52c7b834e052b60 | [
"JavaScript",
"Markdown"
] | 2 | JavaScript | arnesten/smartdb-rediscacheprovider | fe2e6f64f8a5045808349cdeda965d759227cd2a | 644936bb9e07aac3efda3cf7dbde72fee7dc3bf2 | |
refs/heads/master | <repo_name>kinwahlai/xcbeautify<file_sep>/Sources/xcbeautify/Version.swift
let version = "0.8.0"
<file_sep>/Sources/xcbeautify/root.swift
import Guaka
import XcbeautifyLib
var rootCommand = Command(
usage: "xcbeautify",
configuration: configuration,
run: execute
)
private func configuration(command: Command) {
command.add(flags: [
.init(shortName: "q",
longName: "quiet",
value: false,
description: "Only print tasks that have warnings or errors.",
inheritable: true),
])
command.add(flags: [
.init(longName: "quieter",
value: false,
description: "Only print tasks that have errors.",
inheritable: true),
])
command.add(flags: [
.init(longName: "is-ci",
value: false,
description: "Print test result too under quiet/quiter flag"),
])
command.add(flags: [
.init(shortName: "v",
longName: "version",
value: false,
description: "Prints the version",
inheritable: true),
])
command.inheritablePreRun = { flags, args in
if let versionFlag = flags.getBool(name: "version"), versionFlag == true {
print("Version: \(version)")
return false
}
return true
}
command.example = """
$ xcodebuild test -project MyApp.xcodeproj -scheme MyApp -destination 'platform=iOS Simulator,OS=12.0,name=iPhone X' | xcbeautify
"""
}
private func execute(flags: Flags, args: [String]) {
let parser = Parser()
let quiet = flags.getBool(name: "quiet") == true
let quieter = flags.getBool(name: "quieter") == true
let isCI = flags.getBool(name: "is-ci") == true
let output = OutputHandler(quiet: quiet, quieter: quieter, isCI: isCI, { print($0) })
while let line = readLine() {
guard let formatted = parser.parse(line: line) else { continue }
output.write(parser.outputType, formatted)
}
if let summary = parser.summary {
print(summary.format())
}
}
| 767dc7d119a20f601758b8b287ae753ad4bdb636 | [
"Swift"
] | 2 | Swift | kinwahlai/xcbeautify | 8b9efca24694e879847fa639280a91ea0ff988e0 | 02278065b89db846e454574fadd148110214f492 | |
refs/heads/master | <repo_name>NguyenVanLamCNTT/Clone-Trello-Nodejs<file_sep>/server/controllers/users.js
const Users = require('../models/users');
const Accounts = require('../models/accounts');
module.exports.findUser = async function (req, res) {
try {
const username = req.params.username;
const account = await Accounts.findOne({username: username});
const user = await Users.findById(account.id_user);
if (!user) throw Error("Error!");
let data = {
username: username,
firstname: user.firstname,
lastname: user.lastname,
phone: user.phone,
email: user.email,
avatar: user.avatar.path
}
res.status(200).json(data);
}catch (err){
res.status(400).json({message: err.toString(),success: false});
}
}
<file_sep>/server/validate/checkToken.validate.js
const Sessions = require('../models/sessions');
module.exports.checkToken = async function(req, res, next){
const token = req.headers['authorization'].split(' ')[1];
const session = await Sessions.findOne({token: token});
if(!session){
return res.status(400).json({message: "You are not logged in", success: false});
}
next();
}
<file_sep>/server/models/accounts.js
const mongoose = require('mongoose');
let accountSchema = new mongoose.Schema({
id_user: String,
username: String,
password: String
})
let Accounts = mongoose.model('Accounts',accountSchema,'accounts');
module.exports = Accounts;
<file_sep>/server/models/users.js
const mongoose = require('mongoose');
let userSchema = new mongoose.Schema({
firstname: String,
lastname: String,
phone: String,
email: String,
avatar: {path: String,cloudId: String}
})
let Users = mongoose.model('Users',userSchema,'users');
module.exports = Users;
<file_sep>/server/models/sessions.js
const mongoose = require('mongoose');
let sessionSchema = new mongoose.Schema({
id_user: String,
token: String,
created: Date
})
let Sessions = mongoose.model('Sessions',sessionSchema,'sessions');
module.exports = Sessions;
<file_sep>/app.js
require('dotenv').config();
const express = require('express');
const mongoose = require('mongoose');
const cors = require('cors');
const app = express();
app.use(cors());
app.use(express.json());
app.get('/',(req,res) => {
res.send('Clone Trello');
});
//Connect mongodb
mongoose.connect(process.env.MONGO_URL,{
useNewUrlParser: true,
useUnifiedTopology: true,
})
.then(()=> console.log('Mongodb connected'))
.catch(err => console.log(err));
//Router
const RegisterRouter = require('./server/routers/api/register');
const LoginRouter = require('./server/routers/api/login');
const LogoutRouter = require('./server/routers/api/logout');
const UserRouter = require('./server/routers/api/users');
app.use('/api/auth/register', RegisterRouter);
app.use('/api/auth/login', LoginRouter);
app.use('/api/auth/logout', LogoutRouter);
app.use('/api/users', UserRouter);
const PORT = process.env.PORT || 3000
app.listen(PORT,() =>{
console.log(`http://localhost:${PORT}`);
})
<file_sep>/server/validate/auth/register.validate.js
module.exports.regexp = function (req,res,next){
const reg_username = /^[a-z0-9]{0,30}$/;
const reg_phone = /^0[389][0-9]{8}$/;
const reg_firstname =/^([A-Z][a-z]{0,20} ){0,6}([A-Z][a-z]{0,20} {0,2})$/;
const reg_lastname = /^([A-Z][a-z]{0,20} ){0,6}([A-Z][a-z]{0,20} {0,2})$/;
const reg_email = /^([a-zA-Z0-9_\.\-])+\@(([a-zA-Z0-9\-])+\.)+([a-zA-Z0-9]{2,4})+$/;
const username = req.body.username;
const password = <PASSWORD>;
const firstname = req.body.firstname;
const lastname = req.body.lastname;
const phone = req.body.phone;
const email = req.body.email;
if(!username || typeof username !== "string" || reg_username.test(username) == false){
return res.status(400).json({message: "Invalid username!"});
}
if(!password || typeof password !== "string" || password.length < 8){
return res.status(400).json({message: "Invalid password!"});
}
if(!firstname || typeof firstname !== "string" || reg_firstname.test(firstname) == false){
return res.status(400).json({message: "Invalid firstname!"});
}
if(!lastname || typeof lastname !== "string" || reg_lastname.test(lastname) == false){
return res.status(400).json({message: "Invalid lastname!"});
}
if(!phone || typeof phone !== "string" || reg_phone.test(phone) == false){
return res.status(400).json({message: "Invalid phone!"});
}
if(!email || typeof email !== "string" || reg_email.test(email) == false){
return res.status(400).json({message: "Invalid email!"});
}
next();
}
const Users = require('../../models/users');
const Accounts = require('../../models/accounts');
module.exports.checkUser = async function(req, res, next){
const user_phone = await Users.findOne({phone: req.body.phone});
const user_email = await Users.findOne({email: req.body.email});
const account = await Accounts.findOne({username: req.body.username});
if(user_email){
return res.status(400).json({message: "email exists!", success: false});
}
if (user_phone){
return res.status(400).json({message: "phone exists!", success: false});
}
if (account){
return res.status(400).json({message: "username exists!", success: false});
}
next();
}
<file_sep>/server/routers/api/logout.js
const express = require('express');
const router = express.Router();
const auth = require('../../controllers/auth');
const validateCheckToken = require('../../validate/checkToken.validate');
router.get('/',validateCheckToken.checkToken,auth.logout);
module.exports = router;
| f1329ad56f5011ac3b4a8692a81b78abf0faedbe | [
"JavaScript"
] | 8 | JavaScript | NguyenVanLamCNTT/Clone-Trello-Nodejs | 5bcadf9554d1e0340f12fa18a2334ea0bb076136 | 4bc19c376c2bf5c7e277d0238f52a522bdd27ae2 | |
refs/heads/master | <repo_name>phantom1996/SOL-India-Website<file_sep>/js/whysolar.js
function auditFn(){
document.getElementById("audit").style.visibility="visible";
document.getElementById("audit").style.display="inline";
document.getElementById("design").style.display="none";
document.getElementById("approval").style.display="none";
document.getElementById("supply").style.display="none";
document.getElementById("installation").style.display="none";
document.getElementById("operation").style.display="none";
document.getElementById("auditimg").style.width="70px";
document.getElementById("auditimg").style.height="70px";
document.getElementById("systemimg").style.width="60px";
document.getElementById("systemimg").style.height="60px";
document.getElementById("approvalimg").style.width="60px";
document.getElementById("approvalimg").style.height="60px";
document.getElementById("supplyimg").style.width="60px";
document.getElementById("supplyimg").style.height="60px";
document.getElementById("installimg").style.width="60px";
document.getElementById("installimg").style.height="60px";
document.getElementById("maintenanceimg").style.width="60px";
document.getElementById("maintenanceimg").style.height="60px";
}
function designFn(){
document.getElementById("audit").style.display="none";
document.getElementById("design").style.display="inline";
document.getElementById("approval").style.display="none";
document.getElementById("supply").style.display="none";
document.getElementById("installation").style.display="none";
document.getElementById("operation").style.display="none";
document.getElementById("auditimg").style.width="60px";
document.getElementById("auditimg").style.height="60px";
document.getElementById("systemimg").style.width="70px";
document.getElementById("systemimg").style.height="70px";
document.getElementById("approvalimg").style.width="60px";
document.getElementById("approvalimg").style.height="60px";
document.getElementById("supplyimg").style.width="60px";
document.getElementById("supplyimg").style.height="60px";
document.getElementById("installimg").style.width="60px";
document.getElementById("installimg").style.height="60px";
document.getElementById("maintenanceimg").style.width="60px";
document.getElementById("maintenanceimg").style.height="60px";
}
function approvalFn(){
document.getElementById("audit").style.display="none";
document.getElementById("design").style.display="none";
document.getElementById("approval").style.display="inline";
document.getElementById("supply").style.display="none";
document.getElementById("installation").style.display="none";
document.getElementById("operation").style.display="none";
document.getElementById("auditimg").style.width="60px";
document.getElementById("auditimg").style.height="60px";
document.getElementById("systemimg").style.width="60px";
document.getElementById("systemimg").style.height="60px";
document.getElementById("approvalimg").style.width="70px";
document.getElementById("approvalimg").style.height="70px";
document.getElementById("supplyimg").style.width="60px";
document.getElementById("supplyimg").style.height="60px";
document.getElementById("installimg").style.width="60px";
document.getElementById("installimg").style.height="60px";
document.getElementById("maintenanceimg").style.width="60px";
document.getElementById("maintenanceimg").style.height="60px";
}
function supplyFn(){
document.getElementById("audit").style.display="none";
document.getElementById("design").style.display="none";
document.getElementById("approval").style.display="none";
document.getElementById("supply").style.display="inline";
document.getElementById("installation").style.display="none";
document.getElementById("operation").style.display="none";
document.getElementById("auditimg").style.width="60px";
document.getElementById("auditimg").style.height="60px";
document.getElementById("systemimg").style.width="60px";
document.getElementById("systemimg").style.height="60px";
document.getElementById("approvalimg").style.width="60px";
document.getElementById("approvalimg").style.height="60px";
document.getElementById("supplyimg").style.width="70px";
document.getElementById("supplyimg").style.height="70px";
document.getElementById("installimg").style.width="60px";
document.getElementById("installimg").style.height="60px";
document.getElementById("maintenanceimg").style.width="60px";
document.getElementById("maintenanceimg").style.height="60px";
}
function installationFn(){
document.getElementById("audit").style.display="none";
document.getElementById("design").style.display="none";
document.getElementById("approval").style.display="none";
document.getElementById("supply").style.display="none";
document.getElementById("installation").style.display="inline";
document.getElementById("operation").style.display="none";
document.getElementById("auditimg").style.width="60px";
document.getElementById("auditimg").style.height="60px";
document.getElementById("systemimg").style.width="60px";
document.getElementById("systemimg").style.height="60px";
document.getElementById("approvalimg").style.width="60px";
document.getElementById("approvalimg").style.height="60px";
document.getElementById("supplyimg").style.width="60px";
document.getElementById("supplyimg").style.height="60px";
document.getElementById("installimg").style.width="70px";
document.getElementById("installimg").style.height="70px";
document.getElementById("maintenanceimg").style.width="60px";
document.getElementById("maintenanceimg").style.height="60px";
}
function operationFn(){
document.getElementById("audit").style.display="none";
document.getElementById("design").style.display="none";
document.getElementById("approval").style.display="none";
document.getElementById("supply").style.display="none";
document.getElementById("installation").style.display="none";
document.getElementById("operation").style.display="inline";
document.getElementById("auditimg").style.width="60px";
document.getElementById("auditimg").style.height="60px";
document.getElementById("systemimg").style.width="60px";
document.getElementById("systemimg").style.height="60px";
document.getElementById("approvalimg").style.width="60px";
document.getElementById("approvalimg").style.height="60px";
document.getElementById("supplyimg").style.width="60px";
document.getElementById("supplyimg").style.height="60px";
document.getElementById("installimg").style.width="60px";
document.getElementById("installimg").style.height="60px";
document.getElementById("maintenanceimg").style.width="70px";
document.getElementById("maintenanceimg").style.height="70px";
} | 8a7b07cd22eb248830f23d6f8b8f5747aead50e1 | [
"JavaScript"
] | 1 | JavaScript | phantom1996/SOL-India-Website | 507dee587253719e33a81558bd54ff1df544d216 | 0ff09da7db7c66338b6034cd9b2d4b435d459e1e | |
refs/heads/master | <file_sep>import requests as rq
import pandas as pd
## Dados sobre quedas de meteoritos ##
jsondata = rq.get('https://data.nasa.gov/resource/y77d-th95.json').json()
print(jsondata)
df = pd.DataFrame(jsondata)
df.mass[1]
df.mass = pd.to_numeric(df.mass)
df.mass[1]
type(df.mass[0])<file_sep>#import os
#os.chdir('C:/Users/Victor/data_science_projects/metallica_lyrics/codes')
import pandas as pd
import unicodecsv
import stringAux as straux
#############################################
### Part 1 - Creating a metallica subset ####
#############################################
with open('../datasets/songdata.csv', 'rb') as f:
reader = unicodecsv.DictReader(f)
lyrics_list = list(reader)
lyrics_df = pd.DataFrame(lyrics_list)
lyrics_df = lyrics_df.drop(columns = 'link') # this column won't be necessary
# the following function returns a dataframe provided that the artist is metallica
metallica_df = lyrics_df.loc[lyrics_df['artist'] == 'Metallica']
###########################################
### Part 2 - Storing all words in a set ###
###########################################
metallica_list = list(metallica_df['text'])
all_words = straux.generate_string_list(metallica_list)
###########################################
### Part 3 - Removing clutter from data ###
###########################################
all_words = straux.remove_verses(all_words)
all_words = straux.remove_chorus(all_words)
all_words = straux.clean_strings(all_words)
all_words = straux.remove_empty_elements(all_words)
###########################################
### Part 4 - Creating cleaned dataframe ###
###########################################
words_df = pd.DataFrame(all_words).rename(columns = {0: 'word'})
words_df = words_df['word'].value_counts()
most_frequent_words = words_df.iloc[0:50]
##############################################
### Part 5 - Plotting and configuring plot ###
##############################################
words_plot = most_frequent_words.plot.barh(x='Index', y='word',
sort_columns=True, figsize=(12,8),
title='50 most frequent words in examined Metallica\'s lyrics')
words_plot.set(xlabel='Count', ylabel='Words')
words_plot.invert_yaxis()
# The following for loop is to put the value aside each bar for clearer visualization
# https://stackoverflow.com/questions/23591254/python-pandas-matplotlib-annotating-labels-above-bar-chart-columns
# http://robertmitchellv.com/blog-bar-chart-annotations-pandas-mpl.html
for i in words_plot.patches:
# the +0.6 in the y position is to attempt to centralize it (it's +0.6 because the y axis is inverted)
words_plot.annotate(str(i.get_width()), xy=(i.get_width(), i.get_y()+0.6), fontsize=8)
#######################################
### Part 6 - Exporting plot as pdf ###
#######################################
fig = words_plot.get_figure()
fig.savefig('../plot.pdf')<file_sep># -*- coding: utf-8 -*-
import pandas as pd
mat = pd.read_csv('data_science_projects/bootstrap sampling/student-mat.csv')
#por = pd.read_csv('data_science_projects/bootstrap sampling/student-por.csv')
mat = mat.drop(columns=['school', 'address', 'reason', 'nursery', 'famsize',
'Pstatus', 'Mjob', 'Fjob', 'traveltime', 'schoolsup',
'famsup', 'guardian', 'paid', 'activities', 'higher',
'internet', 'romantic', 'famrel', 'freetime', 'goout',
'health'])
bs_sample = pd.DataFrame()
N = 1000 # tamanho da minha amostra bs
for i in range(0, N):
bs_sample = bs_sample.append(mat.sample(replace=True))
#mat.describe()
#bs_sample.describe()
mat.mean()
bs_sample.mean()<file_sep># data_science_projects
Repository with some data science projects that I'm currently working on for learning purposes.
<file_sep># -*- coding: utf-8 -*-
import plotly.graph_objs as go
import plotly.offline
tracebr = go.Bar(
x=['1980', '2017'],
y=[0.235, 2.056],
name='Brasil',
marker=dict(
color='rgb(40,200,40)'),
)
traceus = go.Bar(
x=['1980', '2017'],
y=[2.768, 19.390],
name='EUA',
marker=dict(
color='rgb(40,40,200)'),
)
tracech = go.Bar(
x=['1980', '2017'],
y=[0.189, 12.2400],
name='China',
marker=dict(
color='rgb(200,40,40)'),
)
data = [tracebr, traceus, tracech]
layout = go.Layout(
barmode='group',
title='PIB em trilhões (1980 x 2017)'
)
fig = go.Figure(data=data, layout=layout)
plotly.offline.plot(fig, filename='bar.html')
<file_sep># -*- coding: utf-8 -*-
"""
Created on Sat Dec 1 16:20:58 2018
@author: Victor
"""
# This function the elements of a list on another list
def append_elements_on_a_list(list, updated_list):
for element in list:
updated_list.append(element.lower()) # All words must be preferably lowercase
return updated_list
# This function receives a list of strings and returns ALL words (lowercased)
# from across all strings in the list of strings and returns all of these
# words in a single list
def generate_string_list(list_of_strings):
string_list = []
for string in list_of_strings:
# I need this temporary list to store the substrings from the current
# string in the iteration
temp_list = string.split()
string_list = append_elements_on_a_list(temp_list, string_list)
return string_list
def clean_strings(list):
i = 0
for string in list:
list[i] = string.strip(',"!.?()\'[]1234567890-:;')
i += 1
return list
def remove_chorus(list):
while('[chorus]' in list):
list.remove('[chorus]')
return list
def remove_verses(list):
i = 0
for string in list:
if string.startswith('[v'):
del list[i]
elif string.startswith('(v'):
del list[i]
i += 1
return list
def remove_empty_elements(list):
i = 0
for string in list:
if not string:
del list[i]
i += 1
return list<file_sep># -*- coding: utf-8 -*-
import pandas as pd
suicide_rates = pd.read_csv('data_science_projects/brazil_suicides_plot/master.csv')
br_rates = suicide_rates[suicide_rates['country']=='Brazil'][suicide_rates['sex']
== 'male'][suicide_rates['age']=='15-24 years']
br_rates.year = pd.to_datetime(br_rates.year, format='%Y')
br_rates.plot(x='year', y='suicides/100k pop')<file_sep># -*- coding: utf-8 -*-
import plotly.figure_factory as ff
import pandas as pd
import plotly.offline
df = pd.read_csv('C:/Users/Victor/data_science_projects/plotly/band_members.csv')
colors = dict(Vocals = 'rgb(190, 30, 30)',
Keyboards = 'rgb(30, 190, 30)',
Drums = 'rgb(30, 30, 190)',
Bass = 'rgb(190, 30, 190)',
Guitar = 'rgb(190, 190, 30)')
df.Start = pd.to_datetime(df.Start, format='%Y')
df.Finish = pd.to_datetime(df.Finish, format='%Y')
df = df.rename(columns={"Name": "Task"})
fig = ff.create_gantt(df, colors=colors, index_col='Role', show_colorbar=True,
bar_width=0.4, showgrid_x=True, showgrid_y=True,
title='Membros Blind Guardian (1984-2019)')
plotly.offline.plot(fig, filename='gantt.html')
<file_sep>import pandas as pd
from pandasql import sqldf as psql
import sqlite3 as lite
## USANDO UM PANDAS DF COMO UMA TABLE ##
data = {'funcionario': ['Severino', 'Benedito', 'Aparecida', 'Marlene', 'Rosa',
'Sebastião', 'Valentina', '<NAME>', 'Rita'],
'salario': [900, 1100, 840, 1250, 980, 1400, 925, 760, 1900]}
Salaries = pd.DataFrame(data=data)
psql("SELECT * FROM Salaries").head()
## AGORA CRIANDO UMA TABELA E EXPORTANDO PARA UM PANDAS DF
con = lite.connect('test.db')
cur = con.cursor()
cur.execute("CREATE TABLE music(album TEXT, artista TEXT, lançamento TEXT)")
cur.execute("INSERT INTO music VALUES('Walls of Jericho', 'Helloween', '1985')")
cur.execute("INSERT INTO music VALUES('Visions', 'Stratovarius', '1997')")
cur.execute("INSERT INTO music VALUES('Once', 'Nightwish', '2004')")
albums_df = pd.read_sql_query("SELECT * FROM music", con)<file_sep># -*- coding: utf-8 -*-
import xarray as xr
#Além do xarray, é necessário instalar netcdf4
# International Comprehensive Ocean-Atmosphere Data Set da década de 1700
# from https://data.nodc.noaa.gov/cgi-bin/iso?id=gov.noaa.nodc:ICOADS-netCDF
ds = xr.open_dataset('../nc_file/ICOADS_R3.0.0_1700-09.nc')
df = ds.to_dataframe() | 0e07376a530389605c88dad8ed03a361637a5f54 | [
"Markdown",
"Python"
] | 10 | Python | victoraccete/data_science_projects | 42b7e8758d7e4fa04d4675d73eb041274a030662 | 606fc232f2264d1448fd70e6c9983081dccacd5e | |
refs/heads/master | <repo_name>velaraptor/nba_mvp_metric<file_sep>/README.md
NBA MVP Metric
==============
By <NAME> and <NAME>
Introduction
===============
At the end of each season, the National Basketball Association designates the most valuable player
(MVP) based on a voting system whereby broadcasters and journalists rank the five best performing players. Points are then assigned inversely with rank for each ballot cast. The rank-point
equivalencies are as follows:
<b><center>rank for a given ballot points</b>
1st 10
2nd 7
3rd 5
4th 3
5th 1
</b></center>
The award is given to the player receiving the most points.
In recent years, the MVP has been the subject of much criticism from fans and sports commenta-
tors. Wrote Fansided writer <NAME>: “The NBA fails to concretely define the criteria for the
Maurice Podoloff Trophy winner. The only criterion is that the MVP is awarded annually to the
’most valuable player.’ Value is not defined. Scope is not limited.” Many have also claimed that
the award has become a function of player popularity and media attention rather than performance.
To investigate voting behavior, we examine historical data from 1986-2012, looking at metrics in
several areas: player age, position, individual performance, contribution in the context of a team
and salary. We assume here that player salary functions as a proxy variable for understanding
popularity and overall market demand for a particular player. We narrow our focus to the sub-
set of players receiving positive point counts (typically a handful of players each season). Our
question of interest can then be distilled to: “Among the top-performing players, which character-
istics best predict total points?” Data pertaining to basketball metrics, player age, position and
MVP voting was drawn from www.basketball-reference.com. Player salary data was taken from
www.eskimo.com/~pbender.
Two seasons of data (1998-1999 and 2011-2012) were excluded from our analysis as player negotia-
tions prevented completion of these seasons’ games. The 1987-1988 and 1990-1991 seasons were also
excluded as player salary data could not be found. Total point counts per player were normalized
by the total number of points available since the total number of ballots cast differs from year to
year, giving a response variable that can be interpreted as the proportion of the vote captured by a
particular player. Player salaries were normalized by the cumulative league salary for the particular
year to account for inflation.
After aggregating all of the positive point-receiving players over 23 seasons we are left with a sample
size of n = 374. This method makes two important assumptions. The first is that the tastes and
preferences of the sports commentariat are roughly stable over time. To check the validity of this
assumption, we will examine models for both the full 23 seasons as well as for the most recent 10
seasons (n = 166). The second assumption is that the joint distribution of the covariates for players
in the running for MVP are independent from year to year. This assumption becomes important
as there are many recurring players in our sample.
Page with our results can be seen at http://velaraptor.github.io/nba_mvp_metric/
<b>Explanation of Variables:</b>
PER -- Player Efficiency Rating; a measure of per-minute production standardized such that the league average is 15
TS% -- True Shooting Percentage; a measure of shooting effeciency that takes into account 2-point field goals, 3-point field goals, and free throws
eFG% -- Effective Field Goal Percentage; this statistic adjusts for the fact that a 3-point field goal is worth one more point than a 2-point field goal
FTr -- Free Throw Attempt Rate-Number of FT Attempts Per FG Attempt
3PAr -- 3-Point Attempt Rate-Percentage of FG Attempts from 3-Point Range
ORB% -- Offensive Rebound Percentage; an estimate of the percentage of available offensive rebounds a player grabbed while he was on the floor
DRB% -- Defensive Rebound Percentage; an estimate of the percentage of available defensive rebounds a player grabbed while he was on the floor
TRB% -- Total Rebound Percentage; an estimate of the percentage of available rebounds a player grabbed while he was on the floor
AST% -- Assist Percentage; an estimate of the percentage of teammate field goals a player assisted while he was on the floor
STL% -- Steal Percentage; an estimate of the percentage of opponent possessions that end with a steal by the player while he was on the floor
BLK% -- Block Percentage; an estimate of the percentage of opponent two-point field goal attempts blocked by the player while he was on the floor
TOV% -- Turnover Percentage; an estimate of turnovers per 100 plays
USG% -- Usage Percentage; an estimate of the percentage of team plays used by a player while he was on the floor
ORtg -- Offensive Rating: An estimate of points produced (players) or scored (teams) per 100 possession
DRtg -- Defensive Rating: An estimate of points allowed per 100 possessions
OWS -- Offensive Win Shares; an estimate of the number of wins contributed by a player due to his offense
DWS -- Defensive Win Shares; an estimate of the number of wins contributed by a player due to his defense
WS -- Win Shares; an estimate of the number of wins contributed by a player
WS/48 -- Win Shares Per 48 Minutes; an estimate of the number of wins contributed by a player per 48 minutes (league average is approximately .100)
Copyright 2014 <NAME> & <NAME>
<file_sep>/full data tables by year/cleantomvp.R
a<-read.csv("1980.csv")
b<-read.csv("1981.csv")
c<-read.csv("1982.csv")
d<-read.csv("1983.csv")
e<-read.csv("1984.csv")
f<-read.csv("1985.csv")
g<-read.csv("1986.csv")
h<-read.csv("1987.csv")
i<-read.csv("1988.csv")
j<-read.csv("1989.csv")
k<-read.csv("1990.csv")
l<-read.csv("1991.csv")
m<-read.csv("1992.csv")
n<-read.csv("1993.csv")
o<-read.csv("1994.csv")
p<-read.csv("1995.csv")
q<-read.csv("1996.csv")
r<-read.csv("1998.csv")
s<-read.csv("2000.csv")
t<-read.csv("2001.csv")
u<-read.csv("2002.csv")
v<-read.csv("2003.csv")
w<-read.csv("2004.csv")
x<-read.csv("2005.csv")
y<-read.csv("2006.csv")
z<-read.csv("2007.csv")
aa<-read.csv("2008.csv")
ab<-read.csv("2009.csv")
ac<-read.csv("2010.csv")
ad<-read.csv("2011.csv")
a.1<-a[!(a$Pts.Won==0),]
b.1<-b[!(b$Pts.Won==0),]
c.1<-c[!(c$Pts.Won==0),]
d.1<-d[!(d$Pts.Won==0),]
e.1<-e[!(e$Pts.Won==0),]
f.1<-f[!(f$Pts.Won==0),]
g.1<-g[!(g$Pts.Won==0),]
h.1<-h[!(h$Pts.Won==0),]
i.1<-i[!(i$Pts.Won==0),]
j.1<-j[!(j$Pts.Won==0),]
k.1<-k[!(k$Pts.Won==0),]
l.1<-l[!(l$Pts.Won==0),]
m.1<-m[!(m$Pts.Won==0),]
n.1<-n[!(n$Pts.Won==0),]
o.1<-o[!(o$Pts.Won==0),]
p.1<-p[!(p$Pts.Won==0),]
q.1<-q[!(q$Pts.Won==0),]
r.1<-r[!(r$Pts.Won==0),]
s.1<-s[!(s$Pts.Won==0),]
t.1<-t[!(t$Pts.Won==0),]
u.1<-u[!(u$Pts.Won==0),]
v.1<-v[!(v$Pts.Won==0),]
w.1<-w[!(w$Pts.Won==0),]
x.1<-x[!(x$Pts.Won==0),]
y.1<-y[!(y$Pts.Won==0),]
z.1<-z[!(z$Pts.Won==0),]
aa.1<-aa[!(aa$Pts.Won==0),]
ab.1<-ab[!(ab$Pts.Won==0),]
ac.1<-ac[!(ac$Pts.Won==0),]
ad.1<-ad[!(ad$Pts.Won==0),]
a.1$Pts.Won<-a.1$Pts.Won/sum(a.1$Pts.Won)
b.1$Pts.Won<-b.1$Pts.Won/sum(b.1$Pts.Won)
c.1$Pts.Won<-c.1$Pts.Won/sum(c.1$Pts.Won)
d.1$Pts.Won<-d.1$Pts.Won/sum(d.1$Pts.Won)
e.1$Pts.Won<-e.1$Pts.Won/sum(e.1$Pts.Won)
f.1$Pts.Won<-f.1$Pts.Won/sum(f.1$Pts.Won)
g.1$Pts.Won<-g.1$Pts.Won/sum(g.1$Pts.Won)
h.1$Pts.Won<-h.1$Pts.Won/sum(h.1$Pts.Won)
i.1$Pts.Won<-i.1$Pts.Won/sum(i.1$Pts.Won)
j.1$Pts.Won<-j.1$Pts.Won/sum(j.1$Pts.Won)
k.1$Pts.Won<-k.1$Pts.Won/sum(k.1$Pts.Won)
l.1$Pts.Won<-l.1$Pts.Won/sum(l.1$Pts.Won)
m.1$Pts.Won<-m.1$Pts.Won/sum(m.1$Pts.Won)
n.1$Pts.Won<-n.1$Pts.Won/sum(n.1$Pts.Won)
o.1$Pts.Won<-o.1$Pts.Won/sum(o.1$Pts.Won)
p.1$Pts.Won<-p.1$Pts.Won/sum(p.1$Pts.Won)
q.1$Pts.Won<-q.1$Pts.Won/sum(q.1$Pts.Won)
r.1$Pts.Won<-r.1$Pts.Won/sum(r.1$Pts.Won)
s.1$Pts.Won<-s.1$Pts.Won/sum(s.1$Pts.Won)
t.1$Pts.Won<-t.1$Pts.Won/sum(t.1$Pts.Won)
u.1$Pts.Won<-u.1$Pts.Won/sum(u.1$Pts.Won)
v.1$Pts.Won<-v.1$Pts.Won/sum(v.1$Pts.Won)
w.1$Pts.Won<-w.1$Pts.Won/sum(w.1$Pts.Won)
x.1$Pts.Won<-x.1$Pts.Won/sum(x.1$Pts.Won)
y.1$Pts.Won<-y.1$Pts.Won/sum(y.1$Pts.Won)
z.1$Pts.Won<-z.1$Pts.Won/sum(z.1$Pts.Won)
aa.1$Pts.Won<-aa.1$Pts.Won/sum(aa.1$Pts.Won)
ab.1$Pts.Won<-ab.1$Pts.Won/sum(ab.1$Pts.Won)
ac.1$Pts.Won<-ac.1$Pts.Won/sum(ac.1$Pts.Won)
ad.1$Pts.Won<-ad.1$Pts.Won/sum(ad.1$Pts.Won)
salary<-file.path("http://www.eskimo.com/~pbender/misc",c("salaries86.txt","salaries88.txt","salaries89.txt","salaries91.txt","salaries92.txt","salaries93.txt","salaries94.txt","salaries95.txt","salaries96.txt","salaries97.txt","salaries98.txt","salaries00.txt","salaries01.txt","salaries02.txt","salaries03.txt","salaries04.txt","salaries05.txt","salaries06.txt","salaries07.txt","salaries08.txt","salaries09.txt","salaries10.txt","salaries11.txt"))
salary.1<-lapply(salary,readHTMLTable)
grandtotalmvp<-rbind(g.1,i.1,j.1,l.1,m.1,n.1,o.1,p.1,q.1,r.1,s.1,t.1,u.1,v.1,w.1,x.1,y.1,z.1,aa.1,ab.1,ac.1,ad.1)
grandtotalmvp$totals.Rk<-NULL
grandtotalmvp$First<-NULL
write.csv(grandtotalmvp,file="grandtotalmvp_1.csv")
tenyear<-rbind(t.1,u.1,v.1,w.1,x.1,y.1,z.1,aa.1,ab.1,ac.1,ad.1)
tenyear$totals.Rk<-NULL
tenyear$First<-NULL
write.csv(tenyear,file="tenyearmvp_1.csv")
ridge regression and lasso
l.1<file_sep>/2013_data_code/2013_data.R
library(XML)
library(MASS)
thir<-readHTMLTable("http://www.basketball-reference.com/leagues/NBA_2014_totals.html")
thir.1<-as.data.frame(thir)
thir.adv<-readHTMLTable("http://www.basketball-reference.com/leagues/NBA_2014_advanced.html")
thir.adv.1<-as.data.frame(thir.adv)
change.num.totals<-function(list){
team<-list;
for( i in c(4,6:length(team))){
team[,i]<-as.character(team[,i]);
team[,i]<-as.numeric(team[,i]);
}
return(team)
}
change.num.advanced<-function(list){
team<-list;
for( i in c(4,6:length(team))){
team[,i]<-as.character(team[,i]);
team[,i]<-as.numeric(team[,i]);
}
return(team)
}
thir.1.totals<-change.num.totals(thir.1)
thir.1.adv<-change.num.advanced(thir.adv.1)
delete.row<-function(list){
team<-list;
team.1<-team[-grep("Player",team[,2]),]
return(team.1)
}
thir.1.totals<-delete.row(thir.1.totals)
thir.1.adv<-delete.row(thir.1.adv)
has.star<-function(list){
team<-list;
team[,2]<-as.character(team[,2]);
check.star<-grep("\\*$",team[,2]);
team[check.star,2]<-gsub("\\*$","",team[check.star,2]);
return(team);
}
has.multiple.entry<-function(list){
team<-list;
team<-team[!duplicated(team[,2]),];
return(team);
}
thir.1.totals<-has.star(thir.1.totals)
thir.1.totals<-has.multiple.entry(thir.1.totals)
thir.1.adv<-has.star(thir.1.adv)
thir.1.adv<-has.multiple.entry(thir.1.adv)
nba_2013<-merge(thir.1.totals,thir.1.adv,by.x="totals.Player",by.y="advanced.Player")
fix.columns.ndf<-function(list){
x<-list;
x<-data.frame(x,0);
colnames(x)[55]<-"Pts.Won";
colnames(x)[1]<-"Player";
x$totals.Rk<-NULL;
x$advanced.Rk<-NULL;
x$advanced.Pos<-NULL;
x$advanced.Age<-NULL;
x$advanced.Tm<-NULL;
x$advanced.G<-NULL;
x$advanced.MP<-NULL;
return(x);
}
nba_2013<-fix.columns.ndf(nba_2013)
write.csv(nba_2013,file="nba_2013.csv")
salary<-file.path("http://hoopshype.com/salaries",c("brooklyn.htm","atlanta.htm","boston.htm","charlotte.htm","chicago.htm","cleveland.htm","dallas.htm","denver.htm","detroit.htm","golden_state.htm","houston.htm","indiana.htm","la_clippers.htm","la_lakers.htm","memphis.htm","miami.htm","milwaukee.htm","minnesota.htm","new_orleans.htm","new_york.htm","oklahoma_city.htm","orlando.htm","philadelphia.htm","phoenix.htm","portland.htm","sacramento.htm","san_antonio.htm","toronto.htm","utah.htm","washington.htm"))
sal<-lapply(salary,readHTMLTable)
names.extract<-function(list){
newlist<-list(0)
for(i in 1:30){
names(list[[i]])<-c("a","b","c","d","e","f","sal")
}
for(i in 1:30){
newlist[[i]]<-list[[i]]$sal
}
return(newlist)
}
sal.1<-names.extract(sal)
s<-lapply(sal.1,as.data.frame)
df<-ldply(s,data.frame)
df<-df[,1:2]
delete.row<-function(list){
team<-list;
team.1<-team[-grep("Player",team[,1]),]
return(team.1)
}
df.1<-delete.row(df)
df.1[,1]<-as.character(df.1[,1])
df.1[570,1]<-"Nene"
empty.delete.row<-function(data){
team<-data;
team.1<-team[-grep("^$",team[,1]),]
return(team.1)
}
df.2<-empty.delete.row(df.1)
empty<-function(data){
team<-data;
team.1<-team[-grep("Â",team[,2]),]
return(team.1)
}
df.3<-empty(df.2)
delete.money<-function(data){
team<-data;
team[,2]<-as.character(team[,2]);
check.money<-grep("[[:punct:]]",team[,2]);
team[check.money,2]<-gsub("[[:punct:]]","",team[check.money,2]);
return(team)
}
df.3<-delete.money(df.3)
df.3[,2]<-as.numeric(df.3[,2])
total<-df.3[df.3$V1=="TOTALS:",2]
sum.total<-sum(total)
empty.totals<-function(data){
team<-data;
team.1<-team[-grep("TOTALS:",team[,1]),]
return(team.1)
}
df.3<-empty.totals(df.3)
names(df.3)[2]<-"Salary"
df.3$Salary<-df.3$Salary/sum.total
nba_2013.1<-merge(nba_2013,df.3,by.x="Player",by.y="V1")
fitforward<-lm(Pts.Won ~ advanced.WS + Salary + advanced.USG. +
totals.FT + advanced.TOV. + advanced.TS. + totals.PF + advanced.AST. +
totals.FG + totals.MP + advanced.PER + totals.TOV + advanced.STL. +
totals.PTS,data=mvp)
nba_2014<-nba_2013.1[nba_2013.1$totals.G>=76,]
nba_2014[is.na(nba_2014)]<-0
pre<-predict(fitforward,newdata=nba_2014)
m<-data.frame(nba_2014$Player,nba_2014$totals.G,pre)
top.order<-order(m[,3],decreasing=T)
m[top.order,]
| 586ca91e5a6cd08ce5c19666cba8bce736776f4e | [
"Markdown",
"R"
] | 3 | Markdown | velaraptor/nba_mvp_metric | c012efa934f9287157d43c28ab7ce23426968007 | ba5d4eb79e977d725a6539e431b306e6f3f913c2 | |
refs/heads/master | <repo_name>amoolagundla/OnlineRestaurent<file_sep>/src/pages/login/login.ts
import { Platform} from 'ionic-angular';
import {Component} from '@angular/core';
import {NavController} from 'ionic-angular';
import {HomePage} from "../home/home";
import {AuthenticationService} from "../../services/login-service";
import {ValuesService} from "../../services/ValuesService";
import { LoadingController } from 'ionic-angular';
import { AlertController } from 'ionic-angular';
import {RegisterPage} from "../register/register";
import {Facebook, Google} from "ng2-cordova-oauth/core";
import {OauthCordova} from 'ng2-cordova-oauth/platform/cordova';
import 'rxjs/add/operator/catch';
import 'rxjs/add/operator/mergeMap';
/*
Generated class for the LoginPage page.
See http://ionicframework.com/docs/v2/components/#navigation for more info on
Ionic pages and navigation.
*/
@Component({
selector: 'page-login',
templateUrl: 'login.html'
})
export class LoginPage {
private oauth: OauthCordova = new OauthCordova();
private facebookProvider: Facebook = new Facebook({
clientId: "1828020577450397",
appScope: ["email"]
});
private GoogleProvider: Google = new Google({
clientId: "406482357619-hmp0u9j36fst2dt9mhj8nefe8ked5rlb.apps.googleusercontent.com",
appScope: ["email"]
})
public username:string;
public token:string;
public loading :any= this.loadingCtrl.create({
content: "Please wait...",
dismissOnPageChange: true
});
public password:string;
constructor(public nav: NavController,
private authenticationService: AuthenticationService,
public loadingCtrl: LoadingController,
public valuesService: ValuesService,
public alertCtrl: AlertController,public platform:Platform)
{
this.platform = platform;
localStorage.removeItem("UserInfo");
localStorage.removeItem('currentUser');
}
// go to register page
register() {
this.nav.setRoot(RegisterPage);
}
public launch(url:any) {
this.platform.ready().then(() => {
this.oauth.logInVia(this.facebookProvider).then(success => {
console.log("RESULT: " + JSON.stringify(success));
}, error => {
console.log("ERROR: ", error);
});
});
}
public googleLogin(url:any) {
this.platform.ready().then(() => {
this.oauth.logInVia(this.GoogleProvider).then(success => {
console.log("RESULT: " + JSON.stringify(success));
this.nav.setRoot(HomePage);
}, error => {
console.log("ERROR: ", error);
});
});
}
// login and go to home page
login() {
this.loading.present();
this.authenticationService.login(this.username, this.password)
.subscribe(
data => {
this.getUserInfo();
},
error => {
this.loading.dismiss();
this.nav.setRoot(LoginPage);
});
}
getUserInfo()
{
this.valuesService.getAll()
.subscribe(
data => {
this.getCatogories();
localStorage.setItem('UserInfo',JSON.stringify(data));
},
error => {
this.loading.dismiss();
});
}
getCatogories()
{
this.valuesService.getAllCategories()
.subscribe(
data => { this.loading.dismiss();
localStorage.setItem('categories',JSON.stringify(data)); this.nav.setRoot(HomePage);
},
error => {
this.loading.dismiss();
});
}
}
| ef043be3318e66c7aef0e472944a3c05e55e8e70 | [
"TypeScript"
] | 1 | TypeScript | amoolagundla/OnlineRestaurent | 0b73694ac25c99d2c8df8177743ff0fd5048accf | 6020cfb7ee42b6ccde285f56ffcd8e39ef73d178 | |
refs/heads/main | <repo_name>moomenabid/ACP_CAH_Clustering<file_sep>/Projet_Moomen_ABID.R
load("C:/Users/USER/Desktop/Projects/Datamining/poissons.rda")
poissons<-data
library('GGally')
library('ggplot2')
ggpairs(poissons[,5:10])
library(FactoMineR)
res <-PCA(poissons,quanti.sup=c(3:4),quali.sup=c(1:2))#,graph=FALSE)
round(res$eig,digit=2)
barplot(res$eig[,1], main="Eigenvalues", names.arg=1:nrow(res$eig))
abline(h=1,col=2,lwd=2)
# Graphiques des individus et des variables sur le plan factoriel 1-2
plot(res,axes=c(1,2),choix="var")
# Il convient maintenant de projeter les individus
plot(res,axes=c(1,2),choix="ind")
round(res$ind$cos2,digits=3)
round(res$ind$contrib, digits=1)
round(res$var$cos2,digits=3)
round(res$var$contrib,digits=1)
nouv=cbind(poissons[,1:4],log(poissons[,5:10]))
ggpairs(nouv[,5:10])
res2 <-PCA(nouv,quanti.sup=c(3:4),quali.sup=c(1:2))
plot(res2,habillage=2,invisible="quali",title="")
nouv2=cbind(poissons[,1:4],log(poissons[,6:10])-log(poissons[,5]))
res3 <-PCA(nouv2,quanti.sup=c(3:4),quali.sup=c(1:2))
plot(res3,habillage=2,invisible="quali",title="")
# Interprétation avec les variables quantitatives
nouvdes=nouv2[,c(2,5:9)]
des <- catdes(nouvdes, num.var=1)
print(des$quanti$'herbivores',digits=2)
nouv3=nouv2
#nouv3=nouv2[nouv2[,2]!="herbivores",]
res4 <-PCA(nouv3,quanti.sup=c(3:4),quali.sup=c(1:2))
res4$eig
plot(res4,axes=c(1,2),choix="var")
plot(res4,habillage=2,invisible="quali",title="")
library(zoo)
#on remplace les données manquantes par la moyenne de la colonne.
remplacer=nouv3
remplacer$INTE[is.na(remplacer$INTE)] <- mean(remplacer$INTE,na.rm=T)
remplacer$ESTO[is.na(remplacer$ESTO)] <- mean(remplacer$ESTO,na.rm=T)
remplacer$BRAN[is.na(remplacer$BRAN)] <- mean(remplacer$BRAN,na.rm=T)
remplacer$FOIE[is.na(remplacer$FOIE)] <- mean(remplacer$FOIE,na.rm=T)
remplacer$REIN[is.na(remplacer$REIN)] <- mean(remplacer$REIN,na.rm=T)
#clustering
matclust=remplacer[,5:9]
apply(matclust, 2, sd) # écart-type différents
n <- nrow(matclust)
Z <- scale(matclust,center=TRUE,scale=TRUE)*sqrt(n/(n-1)) # on va donc centrer-réduire les données
# hclust avec Ward des données standardisées
d <- dist(Z)
tree <- hclust(d^2/(2*n), method="ward.D") # ici les poids des individus sont 1/n
sum(tree$height) # on retrouve que l'inertie totale est égale à 5 (nombre de variables)
plot(tree, hang=-1, main="CAH de Ward", sub="", xlab="")
# Partition en 4 classes
rect.hclust(tree, 4, border = "blue3")
barplot(sort(tree$height, decreasing = TRUE), main="Hauteurs de l'arbre")
abline(h = 0.4, col="red")
K <- 4
part <- cutree(tree,k=K)
part <- as.factor(part)
levels(part) <- paste("cluster",1:K,sep="")
#Interprétation des classes obtenue en fonction des 5 variables
#quantitatives utilisées et des variables qualitatives espèces
#et régime alimentaire.
des <- catdes(data.frame(part,matclust), num.var=1)
print(des$quanti$'cluster1',digits=2)
print(des$quanti$'cluster2',digits=2)
print(des$quanti$'cluster3',digits=2)
print(des$quanti$'cluster4',digits=2)
des2 <- catdes(data.frame(part,remplacer[,1]), num.var=1)
print(des2$category$'cluster1',digits=2)
print(des2$category$'cluster2',digits=2)
print(des2$category$'cluster3',digits=2)
print(des2$category$'cluster4',digits=2)
des3 <- catdes(data.frame(part,remplacer[,2]), num.var=1)
print(des3$category$'cluster1',digits=2)
print(des3$category$'cluster2',digits=2)
print(des3$category$'cluster3',digits=2)
print(des3$category$'cluster4',digits=2)
# library('missMDA')
# donnee_imput=nouv3[,5:9]
# ncomp <- estim_ncpPCA(donnee_imput)
# res.imp <- imputePCA(donnee_imput, ncp = ncomp$ncp)
# #imputation et qualité
# res.comp<-MIPCA(donnee_imput,ncp = ncomp$ncp,nboot=10)
# plot(res.comp)
<file_sep>/README.md
__# Analyse en composantes principales (ACP)__
__# Classification ascendante hiérarchique__
Des études réalisées sur l’ensemble du territoire de la Guyane Française ont mis en évidence des imprégnations
par le mercure supérieures à la norme OMS dans les cheveux des populations amérindiennes vivant dans la
zone du Haut - Maroni. Cette contamination a été attribuée à la forte consommation par ces populations
de poissons des rivières, ces derniers étant considérés comme les vecteurs privilégiés du métal. En effet,
la contamination mercurielle peut être attribuée pour une part importante aux rejets métalliques liés aux
activités d’orpaillage car le mercure est largement utilisé pour ses propriétés d’amalgation avec les micro
particules d’or.
Les données sont constituées de 80 poissons appartenant à 12 espèces et 4 régimes alimentaires.
Les données sont détaillées selon 5 variables quantitatives:
__MUSC__: qui représente la concentration du mercure dans les muscle.
__INTE__: qui représente la concentration du mercure dans les intestins.
__ESTO__: qui représente la concentration du mercure dans l'etomac.
__FOIE__: qui représente la concentration du mercure dans la foie.
__REIN__: qui représente la concentration du mercure dans les reins.
Il y a aussi des variables qualitatives __ESPECE__ qui désigne l'espèce du poisson et __REGIME__ qui désigne son régime alimentaire.
L'objectif était de classifier ces poissons en 4 classes selon le principe de __classification ascendante hiérarchique de Ward (CAH)__ et puis on a trouvé les caractéristiques de ces
4 classes à travers les variables quantitatives, ensuite on a utilisé ces classes là pour décrire les espèces et savoir les caractéristiques de chaque espèce.
On a donc pu dire enfin pour chaque espèce , les caractéristiques de ses organes en concentration en mercure et on a pu alors donner des recommandations suite à cela.
Par exemple si l'__espèce 1__ est incluse dans la __classe 1__, et la classe 1 est caractérisé par de fortes concentration de mercure dans les intestins, on recommande de ne pas consommer
les intestins lorsqu'on achète des poissons de cette espèce.
Le fichier __Projet_Moomen_Abid.R__ contient le code source de ce projet.
Le fichier __ACP_CAH_Retour.pdf__ contient le rapport qui détaille ce qu'a été fait dans le projet.
Le fichier __Poissons.rda__ contient la source de ce projet.
| abd3518e6d0fdd08f2bdd142cad67975ded05688 | [
"Markdown",
"R"
] | 2 | R | moomenabid/ACP_CAH_Clustering | d325f68c92fe6a7ddfe560db5f83bdbe3e4c0fbe | 0dfad9824e96163c05a4f2879e26c69813b8e298 | |
refs/heads/master | <file_sep># Carded
Cartões digitais interativos.
Quem puder ajudar no código seja bem-vindo para criar issues!
<file_sep>/* Variáveis para substituir título do link*/
var instagram = document.querySelector("#instagram")
var facebook = document.querySelector("#facebook")
var whatsapp = document.querySelector("#whatsapp")
var site = document.querySelector("#site")
var ifood = document.querySelector("#ifood")
var linkedin = document.querySelector("#linkedin")
var maps = document.querySelector("#maps")
var telefone = document.querySelector("#telefone")
/* Links para interação */
var instagramLink = document.querySelector("#instagramLink")
var facebookLink = document.querySelector("#facebookLink")
var whatsappLink = document.querySelector("#whatsappLink")
var siteLink = document.querySelector("#siteLink")
var ifoodLink = document.querySelector("#ifoodLink")
var linkedinLink = document.querySelector("#linkedinLink")
var mapsLink = document.querySelector("#mapsLink")
var telefoneLink = document.querySelector("#telefoneLink") | 848d8a379f11cd825595b549ed715ed80f9a37cf | [
"Markdown",
"JavaScript"
] | 2 | Markdown | PedroRicardoAlmeida/Carded | ea17bc5f8ad74571cc826acb292a9b313b5067d3 | 8c52859b9e2702b991e8246a8ad3e2b0389a598d | |
refs/heads/main | <file_sep>import pandas as pd
import numpy as np
import csv
import random
random.seed(10)
import tqdm
def balanceClass():
for year in tqdm.tqdm(["2011","2012","2013","2014","2015"]):
df = pd.read_csv("../Data/players_"+year+".csv")
df1 = pd.read_csv("../Data/team_"+year+".csv")
assert len(df.index) == len(df1.index) , "check the Files"
assert df1['winner'].sum() == df["Winner"].sum() , "check the files"
ones = df1['winner'].sum()
total = len(df1.index)
delta = ones - (total/2)
print("Imbalance before:" + str(delta))
listRows = []
for i in range(len(df.index)):
if int(df.iloc[i,-1:]) == 1:
listRows.append(i)
random.shuffle(listRows)
for i in range(int(delta)):
#print(i)
rowNumber = listRows[i]
#print(rowNumber)
assert df.loc[rowNumber][-1] == df1.loc[rowNumber][-1] , "check the files"
teamData = df1.loc[rowNumber].to_numpy()
playersData = df.loc[rowNumber].to_numpy()
whoWonInit = df.loc[rowNumber][-1]
#delta = delta- 1
dataPlayers = playersData[:460]
dataPlayersTeam1 = dataPlayers[:230]
dataPlayersTeam2 = dataPlayers[230:460]
dataTeams = teamData[:48]
dataTeamsTeam1 = dataTeams[:24]
dataTeamsTeam2 = dataTeams[24:48]
assert len(dataPlayersTeam2) == len(dataPlayersTeam2) , "Error"
assert len(dataTeamsTeam2) == len(dataTeamsTeam1) , "Error"
newFirstTeam = dataTeamsTeam2
lineAfterSecondTeam = np.append(newFirstTeam,dataTeamsTeam1)
newFirstPlayerTeam = dataPlayersTeam2
lineAfterPlayerSecondTeam = np.append(newFirstPlayerTeam,dataPlayersTeam1)
whoWonInit = not (whoWonInit)
finalTeamLine = np.append(lineAfterSecondTeam,whoWonInit)
finalPlayerLine = np.append(lineAfterPlayerSecondTeam,whoWonInit)
df1.loc[rowNumber] = finalTeamLine
#print(df1.loc[rowNumber])
df.loc[rowNumber] = finalPlayerLine
#print(df1.loc[rowNumber])
#print(df)
df.to_csv("./Data/playersBal_"+year+".csv",index=False)
df1.to_csv("./Data/teamBal_"+year+".csv",index=False)
#print(df['Winner'])
print("Imbalance Now : " + str(int(df["Winner"].sum()-len(df.index)/2)))
#print(len(df.index))
balanceClass()
<file_sep>import numpy as np
import pandas as pd
import requests
from bs4 import BeautifulSoup
import tqdm
from pathlib import Path
data_folder = Path("../Data1/")
#dataDict = dict()
#dataPDict = dict()
def rowchef():
datastr = ["SetsPlayed","AttackKills","AttackErrors","AttackTotalAttempts","AttackPCT","Assists","AssistErrors",
"AssistTotalAttempts","AssistPCT","ServeAces","ServeErrors","ServeTotalAttempts","ServePCT",
"BlockingDig","BallHandlingErrors","BlockSolos","BlockAssists","BlockErrors","ReceptO","ReceptErrors","ReceptPCT",
"Block_Efficiency_Player","Ace_Strength"
]
finalDatastr = []
for i in range (20):
for str1 in datastr:
finalDatastr.append(str1)
finalDatastr.append("Winner")
#print(finalDatastr.shape)
return finalDatastr
def tablechef(soupObj):
dataTable = []
for element in soupObj:
sub_data = []
for sub_element in element:
try:
string = sub_element.text
string = string[:len(string)-1]
sub_data.append(string)
except:
continue
dataTable.append(sub_data)
return pd.DataFrame(data = dataTable)
def weightschef(shape,decay=0.9):
wt = []
wt = np.array(wt,dtype=float)
for i in range(shape):
wt = wt*(decay)
wt = np.append(wt,1)
return wt
import csv
import numpy as np
def dataChef(dataDict,dataPDict,file1name="../Data/links_2011.csv",file2name="../Data/innovators_2011.csv",file3name="../Data/swarriors_2011.csv",decay=0.9):
dflinks = pd.read_csv(file1name)
file1 = open(file2name, 'w', newline='');
file2 = open(file3name,'w',newline='');
writer = csv.writer(file1)
writerP = csv.writer(file2)
writer.writerow(["SetsPlayed","AttackKills","AttackErrors","AttackTotalAttempts","AttackPCT","Assists","AssistErrors",
"AssistTotalAttempts","AssistPCT","ServeAces","ServeErrors","ServeTotalAttempts","ServePCT",
"BlockingDig","BallHandlingErrors","BlockSolos","BlockAssists","BlockErrors","ReceptO","ReceptErrors","ReceptPCT",
"TotalTeamBlocks", "Set_win_ratio","winrate","SetsPlayed","AttackKills","AttackErrors","AttackTotalAttempts","AttackPCT","Assists","AssistErrors",
"AssistTotalAttempts","AssistPCT","ServeAces","ServeErrors","ServeTotalAttempts","ServePCT",
"BlockingDig","BallHandlingErrors","BlockSolos","BlockAssists","BlockErrors","ReceptO","ReceptErrors","ReceptPCT",
"TotalTeamBlocks", "Set_win_ratio", "winrate",
"winner"])
writerP.writerow(rowchef())
#len(dflinks.index)
for i in tqdm.tqdm(range(len(dflinks.index))):
try:
res = requests.get(dflinks.loc[i][0])
soup = BeautifulSoup(res.content,'html.parser')
if "2012" not in file1name:
table = (soup.find_all("table", border=0 ,cellspacing=0 ,cellpadding= 2))[5].find_all('tr')
settable = (soup.find_all("table", border=0 ,cellspacing=0 ,cellpadding= 2))[7].find_all('tr')
table1 = (soup.find_all("table", border=0 ,cellspacing=0 ,cellpadding= 2))[8].find_all('tr')
else :
table = (soup.find_all("table", border=0 ,cellspacing=0 ,cellpadding= 2))[6].find_all('tr')
settable = (soup.find_all("table", border=0 ,cellspacing=0 ,cellpadding= 2))[8].find_all('tr')
table1 = (soup.find_all("table", border=0 ,cellspacing=0 ,cellpadding= 2))[9].find_all('tr')
df=tablechef(table)
#print(list(df.columns))
if len(df.columns)>23:
#print(len(df.columns))
df.drop(df.columns[len(df.columns)-1], axis=1, inplace=True)
dfset = tablechef(settable)
team1 = str(dfset.loc[1][0]).strip()
team2 = str(dfset.loc[3][0]).strip()
df1 = tablechef(table1)
if len(df1.columns)>23:
df1.drop(df1.columns[len(df1.columns)-1], axis=1, inplace=True)
team1_score = (str(dfset.loc[1][1]).split('('))[1][0]
team1_score = int(team1_score)
team2_score = (str(dfset.loc[3][1]).split('('))[1][0]
team2_score = int(team2_score)
team1Array = []
team2Array = []
if i>0:
if (team1.strip() in dataDict.keys()):
team1Array = np.average(dataDict[team1],0,weightschef(len(dataDict[team1]),decay))
else:
team1Array = np.average(dataDict["goldStandard"],0,weightschef(len(dataDict["goldStandard"]),decay))
if (team2.strip() in dataDict.keys()) :
team2Array = np.average(dataDict[team2],0,weightschef(len(dataDict[team2]),decay))
else:
team2Array = np.average(dataDict["goldStandard"],0,weightschef(len(dataDict["goldStandard"]),decay))
dataArray = np.append(team1Array,team2Array)
dataArraywithPrediction = np.append(dataArray,team2_score > team1_score)
# print(dataArraywithPrediction)
writer.writerow(dataArraywithPrediction)
playerWriter = []
team1PlayerCount = 0
team2PlayerCount = 0
for tuples in dataPDict.keys():
if tuples[0]==team1:
playerWriter.append(np.average(dataPDict[tuples],0,weightschef(len(dataPDict[tuples]),decay)))
team1PlayerCount += 1
if team1PlayerCount==10:
break
while team1PlayerCount<10:
playerWriter.append(np.average(dataPDict[("goldStandard","X","X")],0,weightschef(len(dataPDict[("goldStandard","X","X")]),decay)))
team1PlayerCount+=1
for tuples in dataPDict.keys():
if tuples[0]==team2:
playerWriter.append(np.average(dataPDict[tuples],0,weightschef(len(dataPDict[tuples]),decay)))
team2PlayerCount += 1
if team2PlayerCount==10:
break
while team2PlayerCount<10:
playerWriter.append(np.average(dataPDict[("goldStandard","X","X")],0,weightschef(len(dataPDict[("goldStandard","X","X")]),decay)))
team2PlayerCount+=1
#print(len(playerWriter))
playerWriterFlat = np.array(playerWriter,dtype=float).flatten()
playerWriterFlatWithWinner = np.append(playerWriterFlat,dataArraywithPrediction[-1])
writerP.writerow(playerWriterFlatWithWinner)
set_win_ratio1 = str(team1_score/(team1_score + team2_score))
set_win_ratio2 = str(team2_score/(team1_score + team2_score))
line = (df.loc[df[1].str.contains('Total',na=False)]).to_numpy()
lineClean = np.delete(line,0)
lineClean1 = np.delete(lineClean,0)
lineClean2 = np.append(lineClean1,(str(df.iloc[-1][1]).split())[3])
#print(lineClean2)
lineClean3 = np.append(lineClean2 , set_win_ratio1)
#(lineClean3)
line1 = (df1.loc[df1[1].str.contains('Total',na=False)]).to_numpy()
line1Clean = np.delete(line1,0)
line1Clean1 = np.delete(line1Clean,0)
#---------CHANGES
line1Clean2 = np.append(line1Clean1,(str(df1.iloc[-1][1]).split())[3])
#---------CHANGES
line1Clean3 = np.append(line1Clean2 , set_win_ratio2)
line2 = np.append(lineClean3,line1Clean3)
#print(line2)
lineAfterTeam2 = np.insert(line2,0,team2);
lineAfterTeam1 = np.insert(lineAfterTeam2,0,team1);
newLine1 = np.append(lineAfterTeam1 ,(str(dfset.loc[1][1]).split('('))[1][0])
newLine2 = np.append(newLine1,(str(dfset.loc[3][1]).split('('))[1][0])
winnerLine = np.append(newLine2 ,int(int(newLine2[len(newLine2)-1]) >
int(newLine2[len(newLine2)-2]))) #team2 wins implies 1
#---------CHANGES
data1 = np.append(winnerLine[2:25],int(winnerLine[-1]==0))
data1 = data1.astype(float)
data2 = np.append(winnerLine[25:48],int(winnerLine[-1]==1))
data2 = data2.astype(float)
dataDict.setdefault(team1.strip(), []).append(data1)
dataDict.setdefault(team2.strip(), []).append(data2)
dataDict.setdefault("goldStandard",[]).append(data1)
dataDict.setdefault("goldStandard",[]).append(data2)
for k in range(len(df.index)-3):
if k<2:
continue
player = (df.loc[k]).to_numpy()
playerStats = player[2:].astype(float)
if (playerStats[13]+playerStats[15]+playerStats[16]+playerStats[14]+playerStats[17])==0:
Block_Efficiency=0
else:
Block_Efficiency = (playerStats[13]+playerStats[15]+playerStats[16]-playerStats[14]-playerStats[17])/(playerStats[13]+playerStats[15]+playerStats[16]+playerStats[14]+playerStats[17])
if playerStats[11]==0:
ace_strength =0
else:
ace_strength = playerStats[9]/playerStats[11]
playerStatsAfterBlockEngineering = np.append(playerStats,Block_Efficiency)
playerStatsAfterAceEngineering = np.append(playerStatsAfterBlockEngineering,ace_strength)
dataPDict.setdefault((team1.strip(),player[0].strip(),player[1].strip()),[]).append(playerStatsAfterAceEngineering)
dataPDict.setdefault(("goldStandard","X","X"),[]).append(playerStatsAfterAceEngineering)
for k in range(len(df1.index)-3):
if k<2:
continue
player = (df1.loc[k]).to_numpy()
playerStats = player[2:].astype(float)
if (playerStats[13]+playerStats[15]+playerStats[16]+playerStats[14]+playerStats[17])==0:
Block_Efficiency=0
else:
Block_Efficiency = (playerStats[13]+playerStats[15]+playerStats[16]-playerStats[14]-playerStats[17])/(playerStats[13]+playerStats[15]+playerStats[16]+playerStats[14]+playerStats[17])
# --- Ace Strength
if playerStats[11]==0:
ace_strength =0
else:
ace_strength = playerStats[9]/playerStats[11]
#----- MADE CHANGES
playerStatsAfterBlockEngineering = np.append(playerStats,Block_Efficiency)
playerStatsAfterAceEngineering = np.append(playerStatsAfterBlockEngineering,ace_strength)
dataPDict.setdefault((team2.strip(),player[0].strip(),player[1].strip()),[]).append(playerStatsAfterAceEngineering)
dataPDict.setdefault(("goldStandard","X","X"),[]).append(playerStatsAfterAceEngineering)
except:
#print(str(i) + " has an ERROR")
pass #LINK has errors
file1.close()
def scrapeData(decay=0.9):
for i in ["2011","2012","2013","2014","2015"]:
print(i+" will be scraped now.")
dataDict = dict()
dataPDict = dict()
dataChef(dataDict,dataPDict,"../Data/links_"+i+".csv","../Data/team_"+i+".csv","../Data/players_"+i+".csv",decay)
if __name__ == "__main__":
scrapeData()
<file_sep>import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
from scipy import stats
from sklearn.linear_model import LogisticRegression
from sklearn.preprocessing import StandardScaler
from sklearn.preprocessing import MinMaxScaler
from sklearn.metrics import confusion_matrix
from sklearn.metrics import roc_auc_score
from sklearn.ensemble import RandomForestClassifier
d_2011 = pd.read_csv('../Data/Players_final_2011.csv')
d_2012 = pd.read_csv('../Data/Players_final_2012.csv')
d_2013 = pd.read_csv('../Data/Players_final_2013.csv')
d_2014 = pd.read_csv('../Data/Players_final_2014.csv')
d_2015 = pd.read_csv('../Data/Players_final_2015.csv')
tunedC = 1
def tuning():
list_years = [d_2011,d_2012,d_2013,d_2014,d_2015]
print("Logistic Regression Tuning for player Data: ")
print("Time Series Split :")
C = np.logspace(-100, 10, 200)
maxroc = 0
minvar = 100000
tunedC = 1
for c in C:
total =[]
for i in range(4):
frames=[]
for j in range(i+1):
frames.append(list_years[j])
train = pd.concat(frames)
test = list_years[i+1]
X_train = train.drop(['Winner'],axis=1)
scaler = StandardScaler()
scaler.fit(X_train)
X_train = scaler.transform(X_train)
y_train = train['Winner']
X_test = test.drop(['Winner'],axis=1)
X_test = scaler.transform(X_test)
y_test = test['Winner']
LR = LogisticRegression(C=c,random_state=6,solver='liblinear',max_iter=100000).fit(X_train, y_train)
y_preds = LR.predict(X_test)
confusion_matrix(y_test,y_preds)
roc = roc_auc_score(y_test,y_preds)
total.append(roc)
if(np.var(total)<minvar and np.mean(total)>0.5):
minvar = np.var(total)
print(minvar)
tunedC = c
# if(np.mean(total))>maxStat:
# maxStat = np.mean(total)
# print(maxStat)
# tunedC = c
print("FINAL Results :")
print("C : " + str(tunedC))
#print("RUCAUC : "+ str(maxStat))
def main():
print("{} shape: {}".format(2011,d_2011.shape))
print("{} shape: {}".format(2012,d_2012.shape))
print("{} shape: {}".format(2013,d_2013.shape))
print("{} shape: {}".format(2014,d_2014.shape))
print("{} shape: {}".format(2014,d_2015.shape))
tuning()
list_years = [d_2011,d_2012,d_2013,d_2014,d_2015]
print("Logistic Regression: ")
total =0
for i in range(4):
frames=[]
for j in range(i+1):
frames.append(list_years[j])
train = pd.concat(frames)
test = list_years[i+1]
X_train = train.drop(['Winner'],axis=1)
scaler = StandardScaler()
scaler.fit(X_train)
X_train = scaler.transform(X_train)
y_train = train['Winner']
X_test = test.drop(['Winner'],axis=1)
X_test = scaler.transform(X_test)
y_test = test['Winner']
LR = LogisticRegression(C=tunedC,random_state=6,max_iter=100000,solver='liblinear').fit(X_train, y_train)
y_preds = LR.predict(X_test)
confusion_matrix(y_test,y_preds)
roc = roc_auc_score(y_test,y_preds)
total += roc
print("{} ROC is {}".format(2012+i,roc))
print(total/4)
main()<file_sep>
There is a directory "Data" which holds all the necessary data files generated during scraping, feature enggineering and everything.
This directory has the data scraped when WE scraped from the website.
"Data1" will contain the files that YOU generate while running the code.
We have coded the .py files in such a way that they consume the data from "Data" but write files in directory "Data1".
This is done to maintain uniformity in results as NCAA links time out unpredictably and the data is minutely dynamic in Nature.
For eg., our current dataset has data worth 299 matches. But it is possible, that due to some server issues/timeout errors, while you are executing the scrape
code, only 297 matches get scraped. Hence, this was necessary. This discrepancy is very small but we chose to consume data from the original directory - "Data"
so that you are able to reproduce the same results. We ensure you that same data would be generated via the scrape code, given the website acts the same.
If you want to check results for the newly generated data, please change the "Data" to "Data1" in the .py files. The files have a var named "data_folder", so if you change it, it will directly take the files from "Data1".
\src - contains all the .py files
\Data - contains original data files
\Data1 - files would get created here if you run the .py files
Run getData.py which is a driver for all the scraper functions.
First we scrape the links using scrape_links_from_webpage which generates csv files having all the matchwise hyper-links.
Then using these links we scrape the match data from NCAA using scrape_table_from_links.py
After that the data is balanced using balanceClass.py [Please find the explanation in the report]
Finally, players_final_dataset_generation.py generates the 3 player representatives data for the teams.
phase1_teamdata_allmodels.py
to run: python phase1_teamdata_allmodels.py
This code runs the 3 models about which we have described about in phase1 of the report and outputs their metric. The data for (2011-2015) has been taken from the Data folder for training.
metrics.py has the code to generate the necessary metrics for each test set.
plot_pruning_graph.py generates the ccp_alpha vs Impurities graph
neuralNetworksplayers, dtplayers and logisticRegplayers.py files have the model training code for the player-wise data.
<file_sep>import requests
from bs4 import BeautifulSoup
import re
import csv
import tqdm
from pathlib import Path
data_folder = Path("../Data/")
def getData(yearNumber):
URL = f"http://fs.ncaa.org/Docs/stats/w_volleyball_champs_records/{str(2010 + yearNumber)}/d1/html/confstat.htm"
page = requests.get(URL)
soup = BeautifulSoup(page.content, 'html.parser')
breaker = [ "ucla-ill.htm", "tex-ore.htm", "wiscpnst.htm", "finals3.htm", "final15.htm"]
printing_on = False
year = 2010 + yearNumber
file_to_write = data_folder/f"links_{str(year)}.csv"
with open(file_to_write, "w", newline = '') as f:
csvwriter = csv.writer(f)
csvwriter.writerow(["URL's"])
for a in soup.find_all('a', href=True):
if(a['href'] == breaker[yearNumber - 1]):
printing_on = True
if(printing_on):
#print(a['href'])
url = f"http://fs.ncaa.org/Docs/stats/w_volleyball_champs_records/{year}/d1/html/"
k = a['href']
b = [url + (str(k))]
csvwriter.writerow(b)
else:
pass
if __name__ == "__main__":
for k in tqdm.tqdm(range(5)):
getData(k+1)<file_sep>from sklearn.tree import DecisionTreeClassifier
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
def plot_pruning_graph(X_train, y_train, clf):
# clf is the model you are using
# Would be declared in the main function like :
# clf = DecisionTreeClassifier(random_state=0, criterion="entropy", ccp_alpha=0.01,
# class_weight='balanced',
# splitter = "random"
path = clf.cost_complexity_pruning_path(X_train, y_train)
ccp_alphas, impurities = path.ccp_alphas, path.impurities
fig, ax = plt.subplots()
ax.plot(ccp_alphas[:-1], impurities[:-1], marker='o', drawstyle="steps-post", color = 'black', ls = '--')
ax.set_xlabel("effective alpha")
ax.set_ylabel("total impurity of leaves")
ax.set_title("Total Impurity vs effective alpha for training set")
#Gets stored
fig.savefig("ImpurityVSalpha.png")<file_sep>import scrape_links_from_webpage as linkscrape
import scrape_table_from_links as tablescrape
import tqdm
import argparse
from balanceClass import balanceClass
import players_final_dataset_generation as makePlayers
def main(decay=0.9):
print("Data Scraping begins")
print("Webscarper is scraping all the Match Links from 2011-2015")
for k in tqdm.tqdm(range(5)):
linkscrape.getData(k+1)
print("All links scarped and dumped into links_$year.csv files . ")
tablescrape.scrapeData(decay)
print("All tables scraped and processed to trainable format.")
print("Lets balance the Data now")
balanceClass()
print("Balancing algorithm finished execution")
makePlayers()
if __name__ == "__main__":
parser = argparse.ArgumentParser(description=' Add the decay')
parser.add_argument('--decay', type=float, default = 0.9,
help='an integer for the decay constant')
args = parser.parse_args()
decay = args.decay
main(decay)
<file_sep>import tensorflow
import keras.layers.advanced_activations
from numpy.random import seed
seed(1)
from sklearn.preprocessing import StandardScaler
tensorflow.random.set_seed(2)
from sklearn.metrics import confusion_matrix
import pandas as pd
from tensorflow.keras import regularizers
from keras.layers.core import Dropout
from tensorflow.keras import layers
from tensorflow.keras import initializers
from sklearn.metrics import roc_auc_score
from numpy.random import seed
seed(1)
d_2011 = pd.read_csv('../Data/Players_final_2011.csv')
d_2012 = pd.read_csv('../Data/Players_final_2012.csv')
d_2013 = pd.read_csv('../Data/Players_final_2013.csv')
d_2014 = pd.read_csv('../Data/Players_final_2014.csv')
d_2015 = pd.read_csv('../Data/Players_final_2015.csv')
print("{} shape: {}".format(2011,d_2011.shape))
print("{} shape: {}".format(2012,d_2012.shape))
print("{} shape: {}".format(2013,d_2013.shape))
print("{} shape: {}".format(2014,d_2014.shape))
print("{} shape: {}".format(2014,d_2015.shape))
def main():
model_nn = keras.Sequential([
keras.layers.Dense(units=20,activation='relu',kernel_regularizer = regularizers.l1_l2(l1=0.0001,l2=0.0001)),
Dropout(0.25),
keras.layers.Dense(units=25,activation='relu',kernel_regularizer = regularizers.l1_l2(l1=0.0001,l2=0.0001)),
Dropout(0.25),
keras.layers.Dense(units=30,activation='relu',kernel_regularizer = regularizers.l1_l2(l1=0.0001,l2=0.0001)),
Dropout(0.25),
keras.layers.Dense(units=1,activation='sigmoid')
])
list_years = [d_2011,d_2012,d_2013,d_2014,d_2015]
roc_l = []
print("ANN Neural Network:")
tunedParameters = {}
# parameters={'batch_size':[4,8],
# 'epochs':[50,100],
# 'optimizer':['adam']}
# maxScore = 0
# for batchSize in parameters['batch_size']:
# for epoch in parameters['epochs']:
# for optim in parameters['optimizer']:
# keras.backend.clear_session()
# model_nn.compile(loss='binary_crossentropy', optimizer=optim, metrics=['accuracy'])
# total =0
# for i in range(4):
# frames=[]
# for j in range(i+1):
# frames.append(list_years[j])
# train = pd.concat(frames)
# test = list_years[i+1]
# X_train = train.drop(['Winner'],axis=1)
# scaler = StandardScaler()
# scaler.fit(X_train)
# X_train = scaler.transform(X_train)
# y_train = train['Winner']
# X_test = list_years[i+1].drop(['Winner'], axis = 1)
# X_test = scaler.transform(X_test)
# y_test = list_years[i+1]['Winner']
# history = model_nn.fit(X_train,y_train,epochs=epoch,batch_size= batchSize,shuffle = False)
# y_preds = model_nn.predict(X_test)
# for i in range(len(y_preds)):
# if y_preds[i]>=0.5:
# y_preds[i]=1
# else:
# y_preds[i]=0
# confusion_matrix(y_test,y_preds)
# roc = roc_auc_score(y_test,y_preds)
# roc_l.append(roc)
# total += roc
# # for i in range(4):
# # print("{} ROC is {}".format(2012+i,roc_l[i]))
# print("Average: {}".format(total/4))
# if total/4 > maxScore:
# maxScore = total/4
# print(maxScore)
# tunedParameters['batch_size'] = batchSize
# tunedParameters['epochs'] = epoch
# tunedParameters['optimizer'] = optim
tunedParameters['batch_size'] = 8
tunedParameters['epochs'] = 50
tunedParameters['optimizer'] = 'adam'
list_years = [d_2011,d_2012,d_2013,d_2014,d_2015]
roc_l = []
cf_l = []
print("ANN Neural Network:")
keras.backend.clear_session()
model_nn.compile(loss='binary_crossentropy', optimizer=tunedParameters['optimizer'], metrics=['accuracy'])
total =0
for i in range(4):
frames=[]
for j in range(i+1):
frames.append(list_years[j])
train = pd.concat(frames)
test = list_years[i+1]
X_train = train.drop(['Winner'],axis=1)
scaler = StandardScaler()
scaler.fit(X_train)
X_train = scaler.transform(X_train)
y_train = train['Winner']
X_test = list_years[i+1].drop(['Winner'], axis = 1)
X_test = scaler.transform(X_test)
y_test = list_years[i+1]['Winner']
history = model_nn.fit(X_train,y_train,epochs=tunedParameters['epochs'],batch_size = tunedParameters['batch_size'] ,shuffle=False)
y_preds = model_nn.predict(X_test)
#y_preds = model_nn.predict(X_train)
for i in range(len(y_preds)):
if y_preds[i]>=0.5:
y_preds[i]=1
else:
y_preds[i]=0
cf_l.append(confusion_matrix(y_test,y_preds))
roc = roc_auc_score(y_test,y_preds)
roc_l.append(roc)
total += roc
for i in range(4):
print("{} ROC is {}".format(2012+i,roc_l[i]))
print(cf_l[i])
print("Average: {}".format(total/4))
if __name__ == "__main__":
main()<file_sep>import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
from scipy import stats
from sklearn.linear_model import LogisticRegression
from sklearn.preprocessing import StandardScaler
from sklearn.preprocessing import MinMaxScaler
from sklearn.metrics import confusion_matrix
from sklearn.metrics import roc_auc_score
from sklearn.ensemble import RandomForestClassifier
from sklearn.tree import DecisionTreeClassifier
from sklearn.metrics import f1_score
from sklearn.metrics import roc_curve
from matplotlib import pyplot
import seaborn as sn
import pandas as pd
import matplotlib.pyplot as plt
from sklearn.metrics import plot_confusion_matrix
%matplotlib inline
def metrics(y_true,y_pred,y_probs):
roc = roc_auc_score(y_true,y_probs)
print("The ROC AUC Score is: {}".format(roc))
cf = confusion_matrix(y_true,y_preds)
print("The Confusion Matrix is:")
print(cf)
f1score = f1_score(y_true, y_preds)
print("The F1 score is: {}".format(f1score))
fpr,tpr,_ = roc_curve(y_true,y_preds)
print("ROC_AUC curve")
pyplot.plot(fpr, tpr, marker='.', label='ROC_AUC')
def metrics_combined(comb_y_true,comb_y_pred,comb_y_probs):
roc =0
f1score =0
roc_l=[]
f1_score_l=[]
cf_total=0
for i in range(4):
roc += roc_auc_score(comb_y_true[i],comb_y_probs[i])
roc_l.append(roc_auc_score(comb_y_true[i],comb_y_probs[i]))
f1score += f1_score(comb_y_true[i],comb_y_pred[i])
f1_score_l.append(f1_score(comb_y_true[i],comb_y_pred[i]))
cf = confusion_matrix(comb_y_true[i],comb_y_pred[i])
cf_total += cf
print("Mean ROC score:{}".format(roc/4))
print("Mean F1 score{}".format(f1score/4))
print("Confusion Matrix for all:")
print(cf_total)
x = list(range(1,5))
plt.plot(x,roc_l,label='ROC')
plt.plot(x,f1_score_l,label='F1_score')
plt.ylabel('Split Vs ROC')
plt.show() | c7c9cf0f3f328d34190e3ba5d8108f07e0fa830c | [
"Markdown",
"Python"
] | 9 | Python | SuhasShanbhogue/Sport-Prediction | ed9e1d693be99bfe6bda665866fe2aba97569a0f | 63f328b04f9d3c673cd5158a97b27910844327c7 | |
refs/heads/master | <repo_name>arif034/Chat-Application<file_sep>/pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>Chat</groupId>
<artifactId>Project</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>war</packaging>
<properties>
<spring.version>4.0.7.RELEASE</spring.version>
<!-- <spring.version>3.2.1.RELEASE</spring.version> -->
<spring.data.version>1.7.0.RELEASE</spring.data.version>
<!-- <spring.data.version>1.3.0.RELEASE</spring.data.version> -->
<thymeleaf.version>2.1.3.RELEASE</thymeleaf.version>
<hsqldb.version>2.3.2</hsqldb.version>
<mysql.version>5.1.33</mysql.version>
<java.version>1.7</java.version>
</properties>
<dependencies>
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.17</version>
</dependency>
<dependency>
<groupId>net.sf.flexjson</groupId>
<artifactId>flexjson</artifactId>
<version>2.0</version>
</dependency>
<dependency>
<groupId>io.socket</groupId>
<artifactId>socket.io-client</artifactId>
<version>1.0.0</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
<version>${spring.version}</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.fasterxml.jackson.core/jackson-core -->
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-core</artifactId>
<version>2.11.3</version>
</dependency>
<dependency>
<groupId>org.webjars</groupId>
<artifactId>jquery</artifactId>
<version>3.3.1</version>
</dependency>
<dependency>
<groupId>org.webjars</groupId>
<artifactId>webjars-locator</artifactId>
<version>0.34</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>org.thymeleaf</groupId>
<artifactId>thymeleaf-spring4</artifactId>
<version>${thymeleaf.version}</version>
<scope>compile</scope>
</dependency>
<!-- JPA -->
<dependency>
<groupId>org.springframework.data</groupId>
<artifactId>spring-data-jpa</artifactId>
<version>${spring.data.version}</version>
</dependency>
<dependency>
<groupId>org.hibernate.javax.persistence</groupId>
<artifactId>hibernate-jpa-2.0-api</artifactId>
<version>1.0.1.Final</version>
</dependency>
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-entitymanager</artifactId>
<version>4.1.9.Final</version>
<scope>runtime</scope>
</dependency>
<!-- <dependency> <groupId>org.hsqldb</groupId> <artifactId>hsqldb</artifactId>
<version>${hsqldb.version}</version> </dependency> -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.21</version>
</dependency>
</dependencies>
<build>
<sourceDirectory>src</sourceDirectory>
<plugins>
<plugin>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.0</version>
<configuration>
<release>11</release>
</configuration>
</plugin>
<plugin>
<artifactId>maven-war-plugin</artifactId>
<version>3.2.1</version>
<configuration>
<warSourceDirectory>WebContent</warSourceDirectory>
</configuration>
</plugin>
<plugin>
<groupId>org.apache.tomcat.maven</groupId>
<artifactId>tomcat7-maven-plugin</artifactId>
<version>2.2</version>
<configuration>
<url>http://192.168.59.103/manager/text</url>
<server>RemoteTomcatServer</server>
<path>/</path>
</configuration>
</plugin>
</plugins>
</build>
</project><file_sep>/WebContent/WEB-INF/queries/mycreateInsertUser.sql
CREATE USER 'arif'@'localhost' IDENTIFIED BY 'arif';
GRANT ALL PRIVILEGES ON * . * TO 'arif'@'localhost';
USE `chat_application`;
select * from user;
ALTER TABLE new_table
RENAME TO user;
SELECT IF( EXISTS(
SELECT *
FROM user
WHERE username ='arif' AND password ='<PASSWORD>' ), 1, 0);
select if(exists(select * from user where username = 'arif' and password = <PASSWORD>'),1,0);
insert into user
VALUES (2,'arif2','#Plotter2', '<EMAIL>', 'India');
INSERT INTO user
VALUES (3,'arif3','#Plotter2', '<EMAIL>', 'India');
CREATE TABLE `user` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`username` varchar(45) DEFAULT NULL,
`password` varchar(45) DEFAULT NULL,
`email` varchar(45) DEFAULT NULL,
`region` varchar(45) DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=6 DEFAULT CHARSET=latin1;<file_sep>/src/com/chat/app/controller/UserController.java
package com.chat.app.controller;
import org.apache.log4j.Logger;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.bind.annotation.RestController;
import com.chat.app.dto.UserDTO;
import com.chat.app.model.User;
import com.chat.app.service.UserService;
@RestController("")
public class UserController
{
private static Logger logger = Logger.getLogger(UserController.class);
@Autowired
private UserService userService;
@RequestMapping(value = "/validate", produces = "application/json; charset=UTF-8")
@ResponseBody
public ResponseEntity<Boolean> validateUser(@RequestBody String json)
{
Boolean bool = new Boolean(true);
UserDTO userDTO = UserDTO.fromJsonToUserDTO(json);
User user = new User();
user.setUsername(userDTO.getUsername());
user.setPassword(<PASSWORD>());
if(userService.validateUser(user.getUsername(), user.getPassword()))
bool = true;
else
bool = false;
return new ResponseEntity<Boolean>(bool, HttpStatus.OK);
}
@RequestMapping(value = "/fetch", produces = "application/json; charset=UTF-8")
@ResponseBody
public User fetchUserById(@RequestParam(value = "id", required = true) Long id)
{
User user = userService.fetchUser(id);
return user;
}
@RequestMapping(value = "/save", produces = "application/json; charset=UTF-8")
@ResponseBody
public void saveNewUser( @RequestBody String json)
{
try
{
UserDTO userDTO = UserDTO.fromJsonToUserDTO(json);
User user = new User();
user.setUsername(userDTO.getUsername());
user.setPassword(<PASSWORD>());
user.setEmail(userDTO.getEmail());
user.setRegion(userDTO.getRegion());
userService.saveNewUser(user);
}catch(Exception e)
{
logger.error("Error occured while regestering entry into database",e);
throw e;
}
}
@RequestMapping(value = "/createnewuser", produces = "application/json; charset=UTF-8")
@ResponseBody
public ResponseEntity<Boolean> createNewUser( @RequestBody String json)
{
Boolean bool = new Boolean(true);
UserDTO userDTO = UserDTO.fromJsonToUserDTO(json);
User user = new User();
user.setFirstname(userDTO.getFirstname());
user.setLastname(userDTO.getLastname());
user.setUsername(userDTO.getUsername());
user.setPassword(<PASSWORD>());
user.setEmail(userDTO.getEmail());
user.setRegion(userDTO.getRegion());
userService.saveNewUser(user);
bool = true;
return new ResponseEntity<Boolean>(bool, HttpStatus.OK);
}
}<file_sep>/src/com/chat/app/controller/SampleClass2.java
package com.chat.app.controller;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.OutputStreamWriter;
import java.io.PrintWriter;
import java.net.ServerSocket;
import java.net.Socket;
import java.util.ArrayList;
import java.util.Arrays;
import com.chat.app.dto.MessageDTO;
public class SampleClass2 {
public static void main(String[] args) throws IOException {
System.out.println("Server is started");
ServerSocket serverSocket = new ServerSocket(8083);
System.out.println("Server is waitting for client request");
Socket s = serverSocket.accept();
BufferedReader br = new BufferedReader(new InputStreamReader(s.getInputStream()));
String message = br.readLine();
System.out.println("S: Client Data ");
String messages = "messaage2";
OutputStreamWriter os = new OutputStreamWriter(s.getOutputStream());
PrintWriter out = new PrintWriter(os);
out.println(messages);
os.flush();
System.out.println("S : data sent from Server to Client ");
String ssa = "String here";
ArrayList<String> al = (ArrayList<String>) Arrays.asList("Hi ","My name is"," Arif");
}
}
<file_sep>/src/com/chat/app/controller/ChatClientController.java
package com.chat.app.controller;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.OutputStreamWriter;
import java.io.PrintWriter;
import java.net.ServerSocket;
import java.net.Socket;
import java.net.UnknownHostException;
import java.util.ArrayList;
import java.util.Arrays;
import org.apache.log4j.Logger;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.bind.annotation.RestController;
import com.chat.app.dto.MessageDTO;
import com.chat.app.dto.UserDTO;
import com.chat.app.model.User;
@RestController("")
public class ChatClientController {
private static Logger logger = Logger.getLogger(UserController.class);
@RequestMapping(value = "/server", produces = "application/json; charset=UTF-8")
@ResponseBody
public String takeNewMessage( @RequestBody String json) throws Exception
{
ServerSocket serverSocket = new ServerSocket(8083);
Socket s = serverSocket.accept();
MessageDTO messageDTO = MessageDTO.fromJsonToMessageDTO(json);
String messages = messageDTO.getMessage();
BufferedReader br = new BufferedReader(new InputStreamReader(s.getInputStream()));
String message = br.readLine();
OutputStreamWriter os = new OutputStreamWriter(s.getOutputStream());
PrintWriter out = new PrintWriter(os);
out.println(messages);
os.flush();
String outString = " S : Message from Client : " + message;
return outString;
}
@RequestMapping(value = "/client", produces = "application/json; charset=UTF-8")
@ResponseBody
public String takeNewMessages( @RequestBody String json) throws Exception
{
String ipAddress = "localhost";
int port = 8083;
String message = "";
String message2 = "";
Socket s = new Socket(ipAddress, port);
MessageDTO messageDTO = MessageDTO.fromJsonToMessageDTO(json);
message = messageDTO.getMessage();
OutputStreamWriter os = new OutputStreamWriter(s.getOutputStream());
PrintWriter out = new PrintWriter(os);
out.println(message);
os.flush();
BufferedReader br = new BufferedReader(new InputStreamReader(s.getInputStream()));
message2 = br.readLine();
String outString = " C : Message from Server : " + message2;
return outString;
}
}
| 599b2c919d6f614dfc5cf3ddbbfff37fc583a4a1 | [
"Java",
"SQL",
"Maven POM"
] | 5 | Maven POM | arif034/Chat-Application | c69969bb652257552f0d7a3fd1441ee7a280940e | 2b7f313ff424a19ab3738d135cad1d4516f0d0fa | |
refs/heads/master | <file_sep>class RenameSongsGenreId < ActiveRecord::Migration
def change
change_table :songs do |t|
t.rename :genre_id, :genre_ids
end
end
end
| 453cac489e7ab4fa8636ebfc4d7ae5490570424c | [
"Ruby"
] | 1 | Ruby | aprietof/playlister-sinatra-v-000 | 432b8ba76cc3ee9214ca0650d6547a4bbf5a5963 | 880ccba4ebffd4583b663b870fd1f19fe3dad676 | |
refs/heads/master | <repo_name>ayodvr/Laravel-POS-application<file_sep>/app/Http/Controllers/SalesController.php
<?php
namespace App\Http\Controllers;
use App\User;
use DB;
use App\Customers;
use App\Product;
use App\Sale;
use App\SaleItem;
use App\SalePayment;
use App\SaleTemp;
use App\Inventory;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Auth;
use Illuminate\Support\Facades\Storage;
use RealRashid\SweetAlert\Facades\Alert;
use Haruncpi\LaravelIdGenerator\IdGenerator;
class SalesController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
$sales = Sale::orderBy('id', 'desc')->first();
//dd($sales);
$customers = Customers::all();
return view('sales.index')->with('customers', $customers);
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
//
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
//dd($request->all());
$this->validate($request,[
'customer_id'=>'required',
'payment_type'=>'required',
'subtotal'=>'required',
'payment'=>'required'
]);
$saleItems = SaleTemp::all();
//dd($saleItems);
if(empty($saleItems->toArray())) {
notify()->error("Please Add some Items to create sale!","Error");
return back();
}
$sales = new Sale;
$sales->customer_id = $request->customer_id;
$sales->user_id = Auth::user()->id;
$sales->payment_type = $request->payment_type;
$discount = $request->discount;
$discount_percent = $request->discount_percent;
$subtotal = $request->subtotal;
$sales->uuid = $this->realUniqId();
$total_discount = $discount + ($discount_percent * $subtotal)/100;
$sales->discount = $total_discount;
$tax = $sales->tax = ($subtotal*0)/100;
$total = $sales->grand_total = $subtotal + $tax - $total_discount;
//insert payment data in the payment table
$payment = $sales->payment = $request->payment;
$dues= $sales->dues = $total - $payment;
if ($dues > 0) {
$sales->status = 0;
} else {
$sales->status = 1;
}
$sales->save();
$customer = Customers::findOrFail($sales->customer_id);
$customer->prev_balance = $customer->prev_balance + $sales->dues;
$customer->update();
if ($request->payment > 0) {
$payment = new SalePayment;
$paid = $payment->payment = $request->payment;
$payment->dues = $total - $paid;
$payment->payment_type = $request->payment;
$payment->comments = $request->comments;
$payment->sale_id = $sales->id;
$payment->user_id = Auth::user()->id;
$payment->save();
}
foreach ($saleItems as $value) {
$saleItemsData = new SaleItem;
$saleItemsData->sale_id = $sales->id;
$saleItemsData->uuid = $sales->uuid;
$saleItemsData->product_id = $value->product_id;
$saleItemsData->cost_price = $value->cost_price;
$saleItemsData->selling_price = $value->selling_price;
$saleItemsData->quantity = $value->quantity;
$saleItemsData->total_cost = $value->total_cost;
$saleItemsData->total_selling = $value->total_selling;
$saleItemsData->save();
//process inventory
$products = Product::find($value->product_id);
if ($products->type == 1) {
$inventories = new Inventory;
$inventories->product_id = $value->product_id;
$inventories->user_id = Auth::user()->id;
$inventories->in_out_qty = -($value->quantity);
$inventories->remarks = 'SALE' . $sales->id;
$inventories->save();
//process product quantity
$products->quantity = $products->quantity - $value->quantity;
$products->save();
}
}
//delete all data on SaleTemp model
SaleTemp::truncate();
$itemssale = SaleItem::where('sale_id', $saleItemsData->sale_id)->get();
// notify()->success("You have successfully added sales!","Success");
return view('sales.complete')
->with('sales', $sales)
->with('saleItemsData', $saleItemsData)
->with('saleItems', $itemssale);
}
public function realUniqId($lenght = 10) {
// uniqid gives 13 chars, but you could adjust it to your needs.
if (function_exists("random_bytes")) {
$bytes = random_bytes(ceil($lenght / 2));
} elseif (function_exists("openssl_random_pseudo_bytes")) {
$bytes = openssl_random_pseudo_bytes(ceil($lenght / 2));
} else {
throw new Exception("no cryptographically secure random function available");
}
return substr(bin2hex($bytes), 0, $lenght);
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show($id)
{
//
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function edit($id)
{
//
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id)
{
//
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id)
{
//
}
}
<file_sep>/app/Http/Controllers/InventoryController.php
<?php
namespace App\Http\Controllers;
use App\Inventory;
use App\Product;
use Illuminate\Http\Request;
class InventoryController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
$products = product::find($id);
$inventories = Inventory::all();
return view('inventory.edit')
->with('products', $products)
->with('inventory', $inventories);
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
//
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
//
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show($id)
{
//
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function edit($id)
{
//
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id)
{
$this->validate($request,[
'in_out_qty' =>'required|numeric|max:999999999',
'remarks'=>'required|max:255'
]);
$products = product::find($id);
$products->quantity = $products->quantity + $request->in_out_qty;
$products->save();
$inventories = new Inventory;
$inventories->products_id = $id;
$inventories->user_id = Auth::user()->id;
$inventories->in_out_qty = $request->in_out_qty;
$inventories->remarks = $request->remarks;
$inventories->save();
notify()->success("You have successfully updated product!","Success");
return view('inventories.edit');
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id)
{
//
}
}
<file_sep>/app/Http/Controllers/ProductsController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Storage;
use RealRashid\SweetAlert\Facades\Alert;
use Haruncpi\LaravelIdGenerator\IdGenerator;
use App\Staffs;
use App\User;
use App\AdminProfile;
use DB;
use App\Customers;
use App\Suppliers;
use App\Product;
use App\Category;
class ProductsController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
$products = Product::orderBy('id','asc')->get();
$categories = Category::pluck('cat_name','id');
//dd($categories);
return view('products.index')->with('products',$products)->with('categories',$categories);
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
$category = Category::orderBy('id','desc')->get();
return view('products.create')->with('category',$category);
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
$this->validate($request,[
'prod_image'=>'image|nullable|max:1999',
'name'=>'required|max:15',
'cat_id'=>'required',
'quantity'=>'required|max:185',
'description'=>'required|max:499',
'cost_price'=>'required',
'selling_price'=>'required'
]);
//Handle file uploads
if($request->hasFile('prod_image')){
//Get file name with the extension
$fileNameWithExt = $request->file('prod_image')->getClientOriginalName();
//Get just filename
$filename = pathinfo($fileNameWithExt,PATHINFO_FILENAME);
//Get just extension
$extension = $request->file('prod_image')->getClientOriginalExtension();
//Filename to store
$fileNameToStore = $filename.'_'.time().'.'.$extension;
//Upload image
$path = $request->file('prod_image')->storeAs('public/prod_image',$fileNameToStore);
} else {
$fileNameToStore = 'noimage.jpg';
}
//dd($request->all());
$product = new Product;
$product->name = $request->name;
$product->prod_image = $fileNameToStore;
$product->quantity = $request->quantity;
$product->description = $request->description;
$product->cost_price = $request->cost_price;
$product->selling_price = $request->selling_price;
$product->cat_id = $request->cat_id;
if($product->save()){
notify()->success("Product Added!","Success");
}
return redirect()->back();
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show($id)
{
//
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function edit($id)
{
$product = Product::findorfail($id);
$categories = Category::all();
//dd($product);
return view('products.edit')->with('product',$product)->with('categories',$categories);
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id)
{
$this->validate($request,[
'prod_image'=>'image|nullable|max:1999',
'name'=>'required|max:15',
'quantity'=>'required|max:185',
'description'=>'required|max:499',
'cost_price'=>'required',
'cat_id'=>'required',
'selling_price'=>'required|max:185'
]);
//Handle file uploads
if($request->hasFile('prod_image')){
//Get file name with the extension
$fileNameWithExt = $request->file('prod_image')->getClientOriginalName();
//Get just filename
$filename = pathinfo($fileNameWithExt,PATHINFO_FILENAME);
//Get just extension
$extension = $request->file('prod_image')->getClientOriginalExtension();
//Filename to store
$fileNameToStore = $filename.'_'.time().'.'.$extension;
//Upload image
$path = $request->file('prod_image')->storeAs('public/prod_image',$fileNameToStore);
} else {
$fileNameToStore = 'noimage.jpg';
}
//dd($request->all());
$product = Product::find($id);
$product->name = $request->name;
$product->quantity = $request->quantity;
$product->description = $request->description;
$product->cost_price = $request->cost_price;
$product->selling_price = $request->selling_price;
$product->cat_id = $request->cat_id;
if($request->hasFile('prod_image')){
$product->prod_image = $fileNameToStore;
}
if($product->save()){
notify()->success("Product Updated!","Success");
return redirect()->route('products.index');
}
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id)
{
$product = Product::findorfail($id);
$product->delete();
notify()->success("Product Deleted!","Success");
return redirect()->back();
}
public function dmp(Request $request){
$id = $request->id;
foreach ($id as $user){
Product::where('id', $user)->delete();
}
notify()->success("Product Deleted!","Success");
return redirect()->back();
}
}
<file_sep>/routes/web.php
<?php
use Illuminate\Support\Facades\Route;
use Illuminate\Support\Facades\Auth;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
// Route::get('/calendar',function (){
// return view('calendar');
// });
Route::get('/', function (){
return view('auth/login');
});
Auth::routes();
Route::post('/pay', 'PaymentController@redirectToGateway')->name('pay');
Route::get('/payment/callback', 'PaymentController@handleGatewayCallback');
// Route::get('/payment-order', function (){
// return view('sales.payments');
// });
Route ::group(['middleware'=>'auth'],function(){
Route::get('changeStatus/{id}','StaffController@changeStatus')->name('changeStatus');
Route::get('/staff_dashboard', 'StaffController@staff_dashboard')->name('staffs.staff_dashboard');
Route::get('/staffs/trashed', 'StaffController@trashed')->name('staffs.trashed');
Route::get('/staffs/kill/{id}', 'StaffController@kill')->name('staffs.kill');
Route::get('/staffs/restore/{id}', 'StaffController@restore')->name('staffs.restore');
Route::resource('/staffs',"StaffController");
});
Route ::group(['middleware'=>'auth'],function(){
Route::get('/dashboard',"AdminProfileController@admin_dashboard")->name('dashboard');
Route::get('/adminprofile/create',"AdminProfileController@create")->name('create');
Route::get('/adminprofile/{id}/edit',"AdminProfileController@edit")->name('edit');
Route::get('/adminprofile/{id}',"AdminProfileController@show")->name('show');
Route::post('/adminprofile/store',"AdminProfileController@store")->name('store');
Route::post('/adminprofile/{id}/update',"AdminProfileController@update")->name('update');
Route::get('/adminprofile/{id}/destroy',"AdminProfileController@destroy")->name('destroy');
Route::get('/register_2',"NewUserController@create")->name('register_2');
Route::post('/store',"NewUserController@user_store")->name('user_store');
});
Route::resource('/sales',"SalesController");
Route::resource('/receiving',"ReceivingController");
Route::resource('/products',"ProductsController");
Route::delete('/delete-products', "ProductsController@dmp")->name('products.dmp');
Route::resource('/customers',"CustomersController");
Route::resource('/suppliers',"SuppliersController");
Route::resource('/categories',"CategoryController");
Route::resource('/inventories',"InventoryController");
Route::resource('/accounts',"AccountController");
Route::resource('/transactions',"TransactionsController");
Route::resource('/expense',"ExpenseController");
Route::resource('/expense_cat',"ExpenseCategoryController");
Route::get('/pdf',"CustomersController@Customer_PDF")->name('customer_pdf');
Route::get('/sales-rep-pdf',"SaleReportController@Sale_PDF")->name('sale_pdf');
Route::resource('api/saletemp','SaleApiController');
Route::resource('api/receivingtemp','ReceivingApiController');
Route::resource('api/prodtemp','ProductsApiController');
Route::resource('/receivingsreport', 'ReceivingsReportController');
Route::resource('/salesreport', 'SaleReportController');
Route::get('login/facebook', 'Auth\LoginController@redirectToFacebook')->name('login.facebook');
Route::get('login/facebook/callback', 'Auth\LoginController@handleFacebookCallback');
Route::get('login/google', 'Auth\LoginController@redirectToGoogle')->name('login.google');
Route::get('login/google/callback', 'Auth\LoginController@handleGoogleCallback');
Route::get('/home', 'HomeController@index')->name('home');
Route::get('forget-password', 'ForgotPasswordController@showForgetPasswordForm')->name('forget.password.get');
Route::post('forget-password', 'ForgotPasswordController@submitForgetPasswordForm')->name('forget.password.post');
Route::get('reset-password/{token}', 'ForgotPasswordController@showResetPasswordForm')->name('reset.password.get');
Route::post('reset-password', 'ForgotPasswordController@submitResetPasswordForm')->name('reset.password.post');
Route::get('login-details','NewUserController@user_store');
Route::post('project/importProject', 'ProjectController@importProject')->name('importProject');
Route::resource('project', 'ProjectController');
<file_sep>/app/Http/Controllers/SaleApiController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Storage;
use RealRashid\SweetAlert\Facades\Alert;
use Haruncpi\LaravelIdGenerator\IdGenerator;
use DB;
use App\Http\Requests\SaleRequest;
use Illuminate\Support\Facades\Input;
use App\Product;
use App\SaleTemp;
use Illuminate\Support\Facades\Response;
use \Auth, \Redirect, \Validator, \Session;
class SaleApiController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
return Response::json(SaleTemp::with('product')->get());
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
//
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
$SaleTemps = new SaleTemp;
$SaleTemps->product_id = $request->product_id;
$SaleTemps->cost_price = $request->cost_price;
$SaleTemps->selling_price = $request->selling_price;
$SaleTemps->quantity = 1;
$SaleTemps->total_cost = $request->cost_price;
$SaleTemps->total_selling = $request->selling_price;
//dd($request->all());
$SaleTemps->save();
return $SaleTemps;
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show($id)
{
//
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function edit($id)
{
//
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id)
{
$SaleTemps = SaleTemp::find($id);
$SaleTemps->quantity = $request->quantity;
// $SaleTemps->total_cost = $request->cost_price;
// $SaleTemps->total_selling = $request->selling_price;
$SaleTemps->save();
return $SaleTemps;
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id)
{
SaleTemp::destroy($id);
}
}
<file_sep>/app/Http/Controllers/ReceivingApiController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Product;
use App\RecevingTemp;
use Illuminate\Support\Facades\Auth;
use Illuminate\Support\Facades\Response;
class ReceivingApiController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
return Response::json(RecevingTemp::with('product')->get());
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
//
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
$type = $request->type;
if ($type == 1) {
$ReceivingTemps = new RecevingTemp;
$ReceivingTemps->product_id = $request->product_id;
$ReceivingTemps->cost_price = $request->cost_price;
$ReceivingTemps->total_cost = $request->total_cost;
$ReceivingTemps->quantity = 1;
$ReceivingTemps->save();
return $ReceivingTemps;
}
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show($id)
{
//
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function edit($id)
{
//
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id)
{
$ReceivingTemps = RecevingTemp::find(3);
$ReceivingTemps->quantity = 5;
$ReceivingTemps->total_cost = 54;
$ReceivingTemps->save();
return $ReceivingTemps;
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id)
{
RecevingTemp::destroy($id);
}
}
<file_sep>/app/Http/Controllers/NewUserController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\User;
use Illuminate\Support\Facades\Hash;
// use Illuminate\Support\Facades\Auth;
use App\Mail\UserMail;
use Mail;
use Illuminate\Support\Str;
use Crypt;
class NewUserController extends Controller
{
public function user_store(Request $request)
{
//dd($request->all());
$this->validate($request,[
'name' => ['required', 'string', 'max:255'],
'email' => ['required', 'string', 'email', 'max:255', 'unique:users'],
// 'usertype' => ['string', 'max:255']
]);
$password = Str::random(6);
$data = [
'name'=> $request->get('name'),
'email'=> $request->get('email'),
'password'=> <PASSWORD>($<PASSWORD>),
'usertype'=> $request->get('usertype')
];
if(User::create($data)){
$email = $data['email'];
$details = [
'name' => $data['name'],
'email'=> $data['email'],
'password' => <PASSWORD>
];
//dd($details['<PASSWORD>']);
Mail::to($email)->send(new UserMail($details));
return response()->json(['success_info'=>'<h6>You have succesfully registered a new user!</h6>']);
}
}
}
<file_sep>/app/Http/Controllers/HomeController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\AdminProfile;
use App\User;
class HomeController extends Controller
{
/**
* Create a new controller instance.
*
* @return void
*/
public function __construct()
{
$this->middleware('auth');
}
/**
* Show the application dashboard.
*
* @return \Illuminate\Contracts\Support\Renderable
*/
public function index()
{
if(Auth()->user()->usertype == 'User'){
return redirect('/staff_dashboard');
}
elseif(Auth()->user()->usertype == 'Admin')
{
return redirect('/dashboard');
}
}
}
<file_sep>/public/js/protractor.js
it('should remove the template directive and css class', function() {
expect($('#template1').getAttribute('ng-cloak')).
toBeNull();
expect($('#template2').getAttribute('ng-cloak')).
toBeNull();
expect($('#template3').getAttribute('ng-cloak')).
toBeNull();
expect($('#template4').getAttribute('ng-cloak')).
toBeNull();
expect($('#template5').getAttribute('ng-cloak')).
toBeNull();
expect($('#template6').getAttribute('ng-cloak')).
toBeNull();
expect($('#template7').getAttribute('ng-cloak')).
toBeNull();
expect($('#template8').getAttribute('ng-cloak')).
toBeNull();
expect($('#template9').getAttribute('ng-cloak')).
toBeNull();
});<file_sep>/app/Staffs.php
<?php
namespace App;
use Illuminate\Database\Eloquent\Model;
// use Illuminate\Database\Eloquent\SoftDeletes;
use Haruncpi\LaravelIdGenerator\IdGenerator;
class Staffs extends Model
{
// use SoftDeletes;
protected $fillable = [
'address','phone','city','photo','date_of_birth','date_employed','department','salary',
'experience','status','slug'
];
// protected $dates = ['deleted_at'];
public function user(){
return $this->belongs('App\User');
}
public function admin_profiles(){
return $this->belongsTo('App\AdminProfile');
}
public function customers(){
return $this->hasMany('App\Customers','id');
}
public function suppliers(){
return $this->hasMany('App\Supplier','id');
}
public static function boot()
{
parent::boot();
self::creating(function ($model) {
$model->uuid= IdGenerator::generate(['table' => 'staffs', 'length' => 10, 'prefix' =>date('ym')]);
});
}
}
<file_sep>/app/Http/Controllers/StaffController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Pagination\Paginator;
use Illuminate\Support\Collection;
use Illuminate\Pagination\LengthAwarePaginator;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Storage;
use Illuminate\Support\Str;
use Haruncpi\LaravelIdGenerator\IdGenerator;
use RealRashid\SweetAlert\Facades\Alert;
use App\Staffs;
use App\User;
use App\AdminProfile;
use App\Customers;
use DB;
class StaffController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function Staff_dashboard()
{
if(Auth()->user()->usertype == 'User'){
return view("staffs.dashboard");
}else
{
notify()->warning("Access Denied!","Caution");
return redirect()->back();
}
}
public function index()
{
// $staffs = Staffs::orderBy('id','asc')->get();
// return view('staffs.index')->with('staffs',$staffs);
// $recs = array();
//$users = DB::table("users")->select("id","name","email","usertype")->get();
// array_push($recs,$users);
// $staffs = DB::table("staffs")->select("user_id","photo","phone","department","experience")->get();
// array_push($recs,$staffs);
// dd($recs);
// return view('staffs.index')->with('recs',$recs);
// $users = DB::table("users")->select("id","name","email","usertype")->get();
// $staffs = DB::table("staffs")->select("user_id","photo","phone","department","experience")->get();
// $merged = $users->merge($staffs);
// $recs = $merged->all();
// dd($recs);
// return view('staffs.index')->with('recs',$recs);
$recs = array();
$staff_rec_arr = array();
$users = DB::table("users")->select("id","name","email","status","usertype")->get();
foreach ($users as $user){
if($user->usertype == "Admin") continue;
$staff_rec = Staffs::where("user_id","=",$user->id)->get();
if(!empty($staff_rec[0])){
$staff_rec_arr["user_id"] = $user->id;
$staff_rec_arr["id"] = $staff_rec[0]->id;
$staff_rec_arr["photo"] = $staff_rec[0]->photo;
$staff_rec_arr["phone"] = $staff_rec[0]->phone;
$staff_rec_arr["name"] = $user->name;
$staff_rec_arr["email"] = $user->email;
$staff_rec_arr["status"] = $user->status;
$staff_rec_arr["usertype"] = $user->usertype;
$staff_rec_arr["department"] = $staff_rec[0]->department;
array_push($recs,$staff_rec_arr);
}else{
$staff_rec_arr["user_id"] = $user->id;
$staff_rec_arr["photo"] = "";
$staff_rec_arr["id"] = "";
$staff_rec_arr["name"] = $user->name;
$staff_rec_arr["email"] = $user->email;
$staff_rec_arr["status"] = $user->status;
$staff_rec_arr["usertype"] = $user->usertype;
$staff_rec_arr["phone"] = "";
$staff_rec_arr["department"] = "";
array_push($recs,$staff_rec_arr);
}
}
//dd($recs);
// $data = $this->paginate($recs);
return view('staffs.index')->with('recs',$recs);
}
// public function paginate($items, $perPage = 3, $page = null, $options = [])
// {
// $pageName = 'page';
// $page = $page ?: (Paginator::resolveCurrentPage() ?: 1);
// //dd($page);
// $items = $items instanceof Collection ? $items : Collection::make($items);
// //dd($items);
// return new LengthAwarePaginator(
// $items->forPage($page, $perPage)->values(),
// $items->count(),
// $perPage,
// $page,
// [
// 'path' => Paginator::resolveCurrentPath(),
// 'pageName' => $pageName,
// ]
// );
// }
public function changeStatus(Request $request, $id)
{
$rec = User::find($request->id);
if ($rec->status == 0) {
# code...
$rec->status=1;
}else{
$rec->status=0;
}
if($rec->save()){
notify()->info(" Status has been changed successfully!","Success!");
}
return redirect()->back();
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
return view('staffs.create');
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
$this->validate($request,[
'address' => 'required',
'phone' => 'required',
'city' => 'required',
'photo' => 'image|nullable|max:1999',
'date_of_birth' => 'required',
'date_employed' => 'required',
'department' => 'required',
'experience' => 'required',
'status' => 'required'
]);
//Handle file uploads
if($request->hasFile('photo')){
//Get file name with the extension
$fileNameWithExt = $request->file('photo')->getClientOriginalName();
//Get just filename
$filename = pathinfo($fileNameWithExt,PATHINFO_FILENAME);
//Get just extension
$extension = $request->file('photo')->getClientOriginalExtension();
//Filename to store
$fileNameToStore = $filename.'_'.time().'.'.$extension;
//Upload image
$path = $request->file('photo')->storeAs('public/photo',$fileNameToStore);
} else {
$fileNameToStore = 'noimage.jpg';
}
//dd($request->all());
$staff = new Staffs;
$staff->address = $request->address;
$staff->user_id = auth()->user()->id;
$staff->user_name = auth()->user()->name;
$staff->user_email = auth()->user()->email;
$staff->user_usertype = auth()->user()->usertype;
$staff->phone = $request->phone;
$staff->city = $request->city;
$staff->photo = $fileNameToStore;
$staff->slug = Str::slug($request->status);
$staff->date_of_birth = $request->date_of_birth;
$staff->date_employed = $request->date_employed;
$staff ->department = $request->department;
$staff->experience = $request->experience;
$staff->status = $request->status;
if($staff->save()){
notify()->success("Profile Created!","Success");
}else{
notify()->error("There was a problem creating your profile!","Error");
}
return redirect('staff_dashboard');
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show($id)
{
$staff = Staffs::find($id);
//dd($staff);
return view('staffs.show')->with('staff',$staff);
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function edit($id)
{
$staff = Staffs::find($id);
//$staff_info = User::find($staff);
//dd($staff_info);
return view('staffs.edit')->with('staff',$staff);
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id)
{
$this->validate($request,[
'address' => 'required',
'phone' => 'required',
'city' => 'required',
'date_of_birth' => 'required',
'date_employed' => 'required',
'department' => 'required',
'experience' => 'required',
'status' => 'required'
]);
if($request->hasFile('photo')){
//Get file name with the extension
$fileNameWithExt = $request->file('photo')->getClientOriginalName();
//Get just filename
$filename = pathinfo($fileNameWithExt,PATHINFO_FILENAME);
//Get just extension
$extension = $request->file('photo')->getClientOriginalExtension();
//Filename to store
$fileNameToStore = $filename.'_'.time().'.'.$extension;
//Upload image
$path = $request->file('photo')->storeAs('public/photo',$fileNameToStore);
}
//dd($request->all());
$staff = Staffs::find($id);
$staff->address = $request->address;
$staff->phone = $request->phone;
$staff->city = $request->city;
$staff->date_of_birth = $request->date_of_birth;
$staff->date_employed = $request->date_employed;
$staff ->department = $request->department;
$staff->experience = $request->experience;
$staff->status = $request->status;
if($request->hasFile('photo')){
$staff->photo = $fileNameToStore;
}
if($staff->save()){
notify()->success("Profile Updated!","Success");
}else{
notify()->error("There was a problem updating your profile!","Error");
}
return redirect()->back();
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id)
{
$user = User::findorfail($id);
$user->delete();
notify()->success("User Deleted!","Success");
return redirect()->back();
}
// public function trashed()
// {
// $staff = Staffs::onlyTrashed()->get();
// return view('staffs.trashed')->with('staff',$staff);
// }
// public function restore($id)
// {
// $staff = Staffs::withTrashed()->where('id',$id)->first();
// $staff->restore();
// alert()->success('Success','Staff Restored!');
// return redirect()->back();
// }
public function kill($id)
{
// $staff = Staffs::withTrashed()->where('id',$id)->first();
// if($staff->photo !== 'noimage.jpg'){
// //Delete image
// Storage::delete('public/photo/'.$staff->photo);
// }
// $delete_act = $staff->forceDelete();
// $staff = Staffs::findorfail($id);
// $delete_act = $staff->delete();
// if($delete_act)
// {
// $del = DB::select("delete from users where id = '$staff->user_id'");
// }
$user = User::findorfail($id);
$delete_act = $user->delete();
if($delete_act)
{
$del = DB::select("delete from staffs where user_id = '$user->id'");
}
notify()->success("Staff Deleted!","Success");
return redirect()->back();
}
}
<file_sep>/app/Http/Controllers/ReceivingController.php
<?php
namespace App\Http\Controllers;
use App\User;
use DB;
use App\Supplier;
use App\Product;
use App\Receving;
use App\Recevingitem;
use App\RecevingPayment;
use App\RecevingTemp;
use App\Inventory;
use Illuminate\Support\Facades\Auth;
use Illuminate\Http\Request;
class ReceivingController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
$receivings = Receving::orderBy('id', 'desc')->first();
//dd($receivings);
$suppliers = Supplier::all();
return view('receiving.index')->with('suppliers', $suppliers);
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
//
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
//dd($request->all());
$this->validate($request,[
'supplier_id'=>'required',
'payment_type'=>'required',
'total'=>'required',
'payment'=>'required'
]);
$receivingItems = RecevingTemp::all();
// dd($receivingItems);
if(empty($receivingItems->toArray())) {
notify()->error("Please Add some Items to create purchase!","Error");
return back();
}
$receiving = new Receving;
$receiving->supplier_id = $request->supplier_id;
$receiving->user_id = Auth::user()->id;
$receiving->payment_type = $request->payment_type;
$payment = $receiving->payment = $request->payment;
$total = $receiving->total = $request->total;
$dues = $receiving->dues = $total - $payment;
$receiving->comments = $request->comments;
if ($dues > 0) {
$receiving->status = 0;
} else {
$receiving->status = 1;
}
$receiving->save();
$supplier = Supplier::findOrFail($receiving->supplier_id);
$supplier->prev_balance = $supplier->prev_balance + $dues;
$supplier->update();
if ($request->payment > 0) {
$payment = new RecevingPayment;
$paid = $payment->payment = $request->payment;
$payment->dues = $total - $paid;
$payment->payment_type = $request->payment;
$payment->comments = $request->comments;
$payment->receiving_id = $receiving->id;
$payment->user_id = Auth::user()->id;
$payment->save();
}
$receivingItemsData=[];
foreach ($receivingItems as $value) {
$receivingItemsData = new RecevingItem;
$receivingItemsData->receiving_id = $receiving->id;
$receivingItemsData->product_id = $value->product_id;
$receivingItemsData->cost_price = $value->cost_price;
$receivingItemsData->quantity = $value->quantity;
$receivingItemsData->total_cost = $value->total_cost;
$receivingItemsData->save();
//process inventory
$products = Product::find($value->product_id);
if ($products->type == 1) {
$inventories = new Inventory;
$inventories->product_id = $value->product_id;
$inventories->user_id = Auth::user()->id;
$inventories->in_out_qty = -($value->quantity);
$inventories->remarks = 'PURCHASE' . $receiving->id;
$inventories->save();
//process product quantity
$products->quantity = $products->quantity - $value->quantity;
$products->save();
}
}
//delete all data on SaleTemp model
RecevingTemp::truncate();
$itemsreceiving = RecevingItem::where('receiving_id', $receivingItemsData->receiving_id)->get();
notify()->success("You have successfully added Purchases!","Success");
return view('receiving.complete')
->with('receiving', $receiving)
->with('receivingItemsData', $receivingItemsData)
->with('receivingItems', $itemsreceiving);
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show($id)
{
//
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function edit($id)
{
//
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id)
{
//
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id)
{
//
}
}
<file_sep>/app/Http/Controllers/CategoryController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Storage;
use RealRashid\SweetAlert\Facades\Alert;
use App\Staffs;
use App\User;
use App\AdminProfile;
use DB;
use App\Customers;
use App\Suppliers;
use App\Product;
use App\Category;
class CategoryController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
$categories = Category::withCount('products')->paginate(10);
//dd($categories);
return view('category.index')->with('categories',$categories);
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
return view('category.create');
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
$this->validate($request,[
'cat_image'=>'image|nullable|max:1999',
'cat_name'=>'required|max:185'
]);
//Handle file uploads
if($request->hasFile('cat_image')){
//Get file name with the extension
$fileNameWithExt = $request->file('cat_image')->getClientOriginalName();
//Get just filename
$filename = pathinfo($fileNameWithExt,PATHINFO_FILENAME);
//Get just extension
$extension = $request->file('cat_image')->getClientOriginalExtension();
//Filename to store
$fileNameToStore = $filename.'_'.time().'.'.$extension;
//Upload image
$path = $request->file('cat_image')->storeAs('public/cat_image',$fileNameToStore);
} else {
$fileNameToStore = 'noimage.jpg';
}
//dd($request->all());
$category = new Category;
$category->cat_name = $request->cat_name;
if($request->hasFile('cat_image')){
$category->cat_image = $fileNameToStore;
}
if($category->save()){
notify()->success("Category Added!","Success");
}
return redirect()->route('categories.index');
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show($id)
{
//
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function edit($id)
{
$category = Category::findorfail($id);
return view('category.edit')->with('category',$category);
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id)
{
$this->validate($request,[
'cat_name'=>'required|max:185'
]);
//Handle file uploads
if($request->hasFile('cat_image')){
//Get file name with the extension
$fileNameWithExt = $request->file('cat_image')->getClientOriginalName();
//Get just filename
$filename = pathinfo($fileNameWithExt,PATHINFO_FILENAME);
//Get just extension
$extension = $request->file('cat_image')->getClientOriginalExtension();
//Filename to store
$fileNameToStore = $filename.'_'.time().'.'.$extension;
//Upload image
$path = $request->file('cat_image')->storeAs('public/cat_image',$fileNameToStore);
} else {
$fileNameToStore = 'noimage.jpg';
}
//dd($request->all());
$category = Category::find($id);
$category->cat_name = $request->cat_name;
if($request->hasFile('cat_image')){
$category->cat_image = $fileNameToStore;
}
if($category->save()){
notify()->success("Category Updated!","Success");
}
return redirect()->route('categories.index');
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id)
{
$category = Category::findorfail($id);
$category->delete();
notify()->success("Category Deleted!","Success");
return redirect()->back();
}
}
<file_sep>/app/Http/Controllers/AccountController.php
<?php
namespace App\Http\Controllers;
use App\Account;
use Illuminate\Http\Request;
class AccountController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
$accounts = Account::orderBy('id','asc')->get();
return view('accounts.index')->with('accounts',$accounts);
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
return view('accounts.create');
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
$this->validate($request,[
'account_no' => 'max:100',
'name' => 'required|max:100',
'company' => 'required|max:100',
'branch' => 'max:100',
'email' => 'max:100',
'balance' => 'required|numeric|max:9999999999'
]);
$account = new Account;
$account->account_no = $request->account_no;
$account->name = $request->name;
$account->company = $request->company;
$account->branch = $request->branch;
$account->user_id = auth()->user()->id;
$account->email = $request->email;
$account->balance = $request->balance;
$account->save();
notify()->success("Account Created!","Success");
return redirect()->back();
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show($id)
{
//
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function edit($id)
{
$account = Account::findorfail($id);
return view('accounts.edit')->with('account',$account);
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id)
{
$this->validate($request,[
'account_no' => 'max:100',
'name' => 'required|max:100',
'company' => 'required|max:100',
'branch' => 'max:100',
'email' => 'max:100',
'balance' => 'required|numeric|max:9999999999'
]);
$account = Account::find($id);
$account->account_no = $request->account_no;
$account->name = $request->name;
$account->company = $request->company;
$account->branch = $request->branch;
$account->email = $request->email;
$account->balance = $request->balance;
$account->save();
notify()->success("Account Updated!","Success");
return redirect()->back();
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id)
{
$account = Account::find($id);
$account->delete();
notify()->success("Account Deleted!","Success");
return redirect()->back();
}
}
<file_sep>/public/assets/js/page/index.js
"use strict";
var chartTextColor = '#96A2B4';
if ($("#message-list").length) {
$("#message-list").css({
height : 400
}).niceScroll();
}
/* chart shadow */
var draw = Chart.controllers.line.prototype.draw;
Chart.controllers.lineShadow = Chart.controllers.line.extend({
draw : function() {
draw.apply(this, arguments);
var ctx = this.chart.chart.ctx;
var _stroke = ctx.stroke;
ctx.stroke = function() {
ctx.save();
ctx.shadowColor = '#00000075';
ctx.shadowBlur = 10;
ctx.shadowOffsetX = 8;
ctx.shadowOffsetY = 8;
_stroke.apply(this, arguments)
ctx.restore();
}
}
});
function monthlySalesChart() {
// $data = document.getElementById('#monthlySalesChart').innerHTML;
var options = {
chart : {
height : 300,
type : 'bar',
foreColor : chartTextColor,
stacked: true,
toolbar : {
show : false,
}
},
colors:['#fab107', '#9C27B0'],
plotOptions : {
bar : {
horizontal : false,
//endingShape : 'rounded',
columnWidth : '55%',
},
},
dataLabels : {
enabled : false
},
stroke : {
show : true,
width : 2,
colors : [ 'transparent' ]
},
series : [{
name : 'Revenue',
data : [ 44, 55, 57, 56, 61, 58, 63, 60, 66, 53, 57, 61 ]
}, {
name : 'Expense',
data : [ 76, 85, 99, 98, 87, 95, 91, 98, 94, 83, 93, 98 ]
} ],
xaxis : {
categories : [ 'Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul',
'Aug', 'Sep', "Oct", "Nov", "Dec" ],
},
yaxis : {
title : {
text : '$ (thousands)'
}
},
fill : {
opacity : 1
},
tooltip : {
y : {
formatter : function(val) {
return "$ " + val + " thousands"
}
}
}
}
var chart = new ApexCharts(document.querySelector("#monthlySalesChart"), options);
chart.render();
}
monthlySalesChart();
function yearlySalesChart() {
var options = {
chart : {
height : 315,
type : 'line',
foreColor : chartTextColor,
shadow : {
enabled : false,
color : '#bbb',
top : 3,
left : 2,
blur : 3,
opacity : 1
},
toolbar : {
show : false,
}
},
stroke : {
width : 7,
curve : 'smooth'
},
series : [ {
name : 'revenue',
data : [ 190, 243, 150, 301, 333, 230, 401, 346, 505, 618, 567 ]
} ],
xaxis : {
type : 'year',
categories : [ '2009', '2010', '2011', '2012', '2013', '2014',
'2015', '2016', '2017', '2018', '2019', ],
},
fill : {
type : 'gradient',
gradient : {
shade : 'dark',
gradientToColors : [ '#f635fd' ],
inverseColors: true,
shadeIntensity : 1,
type : 'horizontal',
opacityFrom : 1,
opacityTo : 1,
stops: [50, 100],
colorStops: ["#b435fd","#fd5035"]
},
},
markers : {
size : 4,
opacity : 0.9,
colors : [ "#FFA41B" ],
strokeColor : "#fff",
strokeWidth : 2,
hover : {
size : 7,
}
},
yaxis : {
min : 0,
max : 700,
title : {
text : '$ (thousands)',
},
}
}
var chart = new ApexCharts(document.querySelector("#yearlySalesChart"), options);
chart.render();
}
yearlySalesChart();
function salesByCountriesChart() {
var chart = document.getElementById('salesByCountriesChart');
var barChart = echarts.init(chart);
barChart.setOption({
tooltip : {
trigger : "item",
formatter : "{a} <br/>{b} : {c} ({d}%)"
},
calculable : !0,
series : [ {
name : "Chart Data",
type : "pie",
radius : "55%",
center : [ "50%", "48%" ],
data : [ {
value : 8500,
name : "India"
}, {
value : 9500,
name : "USA"
}, {
value : 1600,
name : "Cambodia"
}, {
value : 6100,
name : "Germany"
} ]
} ],
color : [ '#575B7A', '#fc544b', '#ffc107', '#50A5D8' ]
});
}
salesByCountriesChart();
<file_sep>/app/Http/Controllers/ChartsController.php
<?php
namespace App\Http\Controllers;
use App\User;
use App\Customers;
use App\Product;
use App\Sale;
use App\Supplier;
use App\Receiving;
use App\Expense;
use App\Staffs;
use Carbon;
use Illuminate\Http\Request;
class ChartsController extends Controller
{
public function index()
{
$items = Product::where('type', 1)->count();
$expenses = Expense::count();
$customers = Customers::count();
$suppliers = Supplier::count();
$receivings = Receiving::count();
$sales = Sale::count();
$employees = Staffs::count();
dd($employees);
// $incomeexpensechart = $this->incomeExpenseChart();
return view('adminprofile.dashboard')
->with('products', $products)
->with('expenses', $expenses)
->with('customers', $customers)
->with('suppliers', $suppliers)
->with('receivings', $receivings)
->with('sales', $sales)
->with('employees', $employees);
// ->with('incomeexpensechart', $incomeexpensechart);
}
// private function getDatesOfWeek()
// {
// $days = array();
// $days[] = date("Y-m-d", strtotime('-14 days'));
// $days[] = date("Y-m-d", strtotime('-13 days'));
// $days[] = date("Y-m-d", strtotime('-12 days'));
// $days[] = date("Y-m-d", strtotime('-11 days'));
// $days[] = date("Y-m-d", strtotime('-10 days'));
// $days[] = date("Y-m-d", strtotime('-9 days'));
// $days[] = date("Y-m-d", strtotime('-8 days'));
// $days[] = date("Y-m-d", strtotime('-7 days'));
// $days[] = date("Y-m-d", strtotime('-6 days'));
// $days[] = date("Y-m-d", strtotime('-5 days'));
// $days[] = date("Y-m-d", strtotime('-4 days'));
// $days[] = date("Y-m-d", strtotime('-3 days'));
// $days[] = date("Y-m-d", strtotime('-2 days'));
// $days[] = date("Y-m-d", strtotime('-1 days'));
// $days[] = date("Y-m-d", strtotime('0 days'));
// return $days;
// }
// public function getMonthListFromDate(Carbon $start)
// {
// $start = $start->startOfMonth();
// $end = Carbon::today()->startOfMonth();
// do{
// $months[$start->format('m-Y')] = $start->format('F Y');
// }while ($start->addMonth() <= $end);
// dd($months);
// return $months;
// }
// public function incomeExpenseChart()
// {
// $monthsOfYear = $this->getMonthListFromDate();
// $incomes = Sale::whereBetween('created_at', [ $monthsOfYear[0], $monthsOfYear[11]])->get();
// $expenses = Receiving::whereBetween('created_at', [ $monthsOfYear[0], $monthsOfYear[11]])->get();
// $chartArray = array();
// foreach ($monthsOfYear as $month) {
// $monthlyincome = "0";
// $monthlyexpense = "0";
// $monthlyincome = Sale::whereBetween('created_at', [ $month ])->count();
// $monthlyexpense = Receiving::whereBetween('created_at', [ $month ])->count();
// $chart = [
// 'y' => $month,
// 'a' => $monthlyexpense,
// 'b'=>$monthlyincome,
// ];
// $chartArray[] = $chart;
// }
// return $chartArray;
// }
}
<file_sep>/app/Product.php
<?php
namespace App;
use Illuminate\Database\Eloquent\Model;
use Haruncpi\LaravelIdGenerator\IdGenerator;
class Product extends Model
{
//Table Name
protected $table = 'products';
//Primary Key
public $primaryKey = 'id';
//Timestamps
public $timestamps = true;
public function sales(){
return $this->hasMany('App\Sales','id');
}
public function inventory()
{
return $this->hasMany('App\Inventory')->orderBy('id', 'DESC');
}
public function categories(){
return $this->belongsTo('App\Category','cat_id');
}
public function saletemp()
{
return $this->hasMany('App\SaleTemp')->orderBy('id', 'DESC');
}
public function saleitem()
{
return $this->hasMany('App\SaleItem');
}
public static function boot()
{
parent::boot();
self::creating(function ($model) {
$model->uuid = IdGenerator::generate(['table' => 'products', 'length' => 10, 'prefix' =>date('ym')]);
});
}
}
<file_sep>/database/seeds/CustomersTableSeeder.php
<?php
use Illuminate\Database\Seeder;
use Illuminate\Support\Facades\DB;
use Faker\Factory as Faker;
class CustomersTableSeeder extends Seeder
{
/**
* Run the database seeds.
*
* @return void
*/
public function run()
{
$faker = Faker::create();
foreach (range(1,20) as $index) {
DB::table('customers')->insert([
'name' => $faker->name,
'email' => $faker->email,
'phone_number' => $faker->e164PhoneNumber,
'address' => $faker->address,
'city' => $faker->city,
'state' => $faker->state,
'zip' => $faker->postcode,
'company_name' => $faker->company,
'account' =>$faker->creditCardType,
'prev_balance' => '20000',
'payment'=>$faker->randomNumber($nbDigits = 5, $strict = true),
'created_at' => date("Y-m-d H:i:s"),
'updated_at' => date("Y-m-d H:i:s")
]);
}
}
}
<file_sep>/app/Http/Controllers/ExpenseCategoryController.php
<?php
namespace App\Http\Controllers;
use App\Expense;
use App\ExpenseCategory;
use Illuminate\Http\Request;
use Illuminate\Support\Str;
class ExpenseCategoryController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
$expense_category = ExpenseCategory::all();
return view('expense_cat.index')->with('expense_categories', $expense_category);
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
return view('expense_cat.create');
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
// dd($request->all());
$this->validate($request,[
'name'=> 'required|max:150',
'description'=> 'required|max:150'
]);
$expense_cat = new ExpenseCategory;
$expense_cat->name = $request->name;
$expense_cat->description = $request->description;
$expense_cat->slug = Str::slug($request->description);
$expense_cat->save();
notify()->success('Expense Category added successfully!','Success');
return redirect()->back();
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show($id)
{
$expense_cat = ExpenseCategory::findOrFail($id);
$expense_cats = ExpenseCategory::all();
return view('expense_cat.show')->with('expense_cat', $expense_cat)
->with('expense_cats',$expense_cats);
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function edit($id)
{
//
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id)
{
//
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id)
{
//
}
}
<file_sep>/app/Customers.php
<?php
namespace App;
use Illuminate\Database\Eloquent\Model;
class Customers extends Model
{
protected $fillable = ['name', 'email', 'phone_number','avatar','address','city','state','zip','company','account','prev_balance','payment','prev_balance'];
public function admin_profiles(){
return $this->belongsTo('App\AdminProfile');
}
public function staffs(){
return $this->belongsTo('App\Staffs');
}
public function user(){
return $this->belongsTo('App\User');
}
}
<file_sep>/app/Http/Controllers/SuppliersController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Storage;
use RealRashid\SweetAlert\Facades\Alert;
use App\Staffs;
use App\User;
use App\AdminProfile;
use DB;
use App\Customers;
use App\Supplier;
class SuppliersController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
$suppliers = Supplier::orderBy('id','asc')->get();
return view('supplier.index')->with('suppliers',$suppliers);
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
return view('supplier.create');
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
$this->validate($request,[
'sup_img'=>'image|nullable|max:1999',
'name'=>'required|max:185',
'email'=>'required|max:100',
'address'=>'required|max:185',
'company_name'=>'required|max:50',
'phone'=>'required|max:20',
'city'=>'required',
'state'=>'required',
'country'=>'required',
'account'=>'max:20',
'prev_balance'=>'max:9999999999|numeric',
'payment'=>'max:9999999999|numeric|nullable' ,
'postal_code'=>'required'
]);
//Handle file uploads
if($request->hasFile('sup_img')){
//Get file name with the extension
$fileNameWithExt = $request->file('sup_img')->getClientOriginalName();
//Get just filename
$filename = pathinfo($fileNameWithExt,PATHINFO_FILENAME);
//Get just extension
$extension = $request->file('sup_img')->getClientOriginalExtension();
//Filename to store
$fileNameToStore = $filename.'_'.time().'.'.$extension;
//Upload image
$path = $request->file('sup_img')->storeAs('public/sup_img',$fileNameToStore);
} else {
$fileNameToStore = 'noimage.jpg';
}
//dd($request->all());
$supplier = new Supplier;
$supplier->name = $request->name;
$supplier->category = $request->category;
$supplier->email = $request->email;
$supplier->phone = $request->phone;
$supplier->sup_img = $fileNameToStore;
$supplier->address = $request->address;
$supplier->company_name = $request->company_name;
$supplier->city = $request->city;
$supplier->state = $request->state;
$supplier->country = $request->country;
$supplier->postal_code = $request->postal_code;
$supplier->comments = $request->comments;
$supplier->payment = $request->payment;
$supplier->prev_balance = $request->prev_balance;
$supplier->account = $request->account;
if($supplier->save()){
notify()->success("Supplier Added!","Success");
}
return redirect()->back();
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show($id)
{
//
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function edit($id)
{
$supplier = Supplier::findorfail($id);
return view('supplier.edit')->with('supplier',$supplier);
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id)
{
$this->validate($request,[
'name'=>'required|max:185',
'email'=>'required|max:100',
'address'=>'required|max:185',
'company_name'=>'required|max:50',
'phone'=>'required|max:20',
'city'=>'required',
'state'=>'required',
'country'=>'required',
'postal_code'=>'required',
'account'=>'max:20',
'prev_balance'=>'max:9999999999|numeric',
'payment'=>'max:9999999999|numeric|nullable'
]);
//Handle file uploads
if($request->hasFile('sup_img')){
//Get file name with the extension
$fileNameWithExt = $request->file('sup_img')->getClientOriginalName();
//Get just filename
$filename = pathinfo($fileNameWithExt,PATHINFO_FILENAME);
//Get just extension
$extension = $request->file('sup_img')->getClientOriginalExtension();
//Filename to store
$fileNameToStore = $filename.'_'.time().'.'.$extension;
//Upload image
$path = $request->file('sup_img')->storeAs('public/sup_img',$fileNameToStore);
} else {
$fileNameToStore = 'noimage.jpg';
}
//dd($request->all());
// dd("am here");
$supplier = Supplier::find($id);
$supplier->name = $request->name;
$supplier->email = $request->email;
$supplier->phone = $request->phone;
$supplier->address = $request->address;
$supplier->company_name = $request->company_name;
$supplier->city = $request->city;
$supplier->state = $request->state;
$supplier->country = $request->country;
$supplier->postal_code = $request->postal_code;
$supplier->comments = $request->comments;
$supplier->payment = $request->payment;
$supplier->prev_balance = $request->prev_balance;
$supplier->account = $request->account;
if($request->hasFile('sup_img')){
$supplier->sup_image = $fileNameToStore;
}
if($supplier->save()){
notify()->success("Supplier Updated!","Success");
}
return redirect()->back();
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id)
{
$supplier = Supplier::findorfail($id);
$supplier->delete();
notify()->success("Supplier Deleted!","Success");
return redirect()->back();
}
}
<file_sep>/README.md
Stock-Management(POS):
A Sales POS web application built with laravel and angular js to cater for every business needs, built with the users in mind ensuring that they get
the maximum satisfation and a wonderful user experience.
Techstack:
-- Laravel 7
-- Angular
-- JS
-- Laravel Mix
-- Bootstrap
-- webpack
-- node Modules
Features:
Stock Adding and Managing
-- Customer Management,
-- Supplier Management,
-- Sales Adding,
-- Purchases Adding,
-- POS(module)
-- Employee management system
-- Sales and purchases report
-- Accounts / Transactions
-- Income and Expenses
-- Invoicing
-- Paystack Payment Integration
-- Run Composer install
-- Run npm install
-- Create a copy of your .env file e.g cp .env.example .env
-- Generate your app encryption key by running the following command
php artisan key:generate
-- Create an empty database for your application
-- In the .env file, add database information to allow Laravel to connect to the database
-- Migrate the database using this command
php artisan migrate
-- Optional: Seed the database using - php artisan db:seed
Then lastly, run your app either by configuring a virtual host or by simply serving it with
- php artisan serve which generates a server for you @ localhost:8000
Enjoy : )
<file_sep>/app/Http/Controllers/AdminProfileController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Support\Facades\Auth;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Storage;
use App\AdminProfile;
use App\Staffs;
use App\User;
use App\Customers;
use App\Product;
use App\Supplier;
use App\Sale;
use App\SaleItem;
use DB;
use Carbon\Carbon;
use Carbon\CarbonPeriod;
use RealRashid\SweetAlert\Facades\Alert;
class AdminProfileController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function admin_dashboard()
{
if(Auth()->user()->usertype == 'Admin'){
$id = Auth::user()->id;
$items = Product::where('type', 1)->count();
$customers = Customers::count();
$staff = Staffs::count();
$sales = DB::table('sales')->where('user_id', $id)->sum('grand_total');
$latest = Sale::orderBy('id','desc')->take(7)->get();
// DB::table('sales')->sum('grand_total')
// $incomeexpensechart = $this->incomeExpenseChart();
return view("adminprofile.dashboard")
->with('items', $items)
->with('staff', $staff)
->with('customers', $customers)
->with('sales', $sales)
->with('latest', $latest);
// ->with('incomeexpensechart', $incomeexpensechart);
}
}
// public function getMonthListFromDate(Carbon $start)
// {
// foreach (CarbonPeriod::create($start, '1 month', Carbon::today()) as $month){
// $months[$months[$month->format('m-Y')]] = $month->format('F Y');
// }
// return $months;
// }
// public function incomeExpenseChart()
// {
// $monthsOfYear = $this->getMonthListFromDate();
// $incomes = Sale::whereBetween('created_at', [ $monthsOfYear[0], $monthsOfYear[11]])->get();
// $expenses = Receiving::whereBetween('created_at', [ $monthsOfYear[0], $monthsOfYear[11]])->get();
// $chartArray = array();
// foreach ($monthsOfYear as $month) {
// $monthlyincome = "0";
// $monthlyexpense = "0";
// $monthlyincome = Sale::whereBetween('created_at', [ $month ])->count();
// $monthlyexpense = Receiving::whereBetween('created_at', [ $month ])->count();
// $chart = [
// // 'y' => $month,
// 'a' => $monthlyexpense,
// 'b'=>$monthlyincome,
// ];
// $chartArray[] = $chart;
// }
// return $chartArray;
// }
public function index()
{
$admins = AdminProfile::all();
return view("adminprofile.index")->with('admins',$admins);
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
return view("adminprofile.create");
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
//dd($request->all());
$this->validate($request,[
'address' => 'required',
'phone' => 'required',
'no_of_staff' => 'required',
'admin_image' => 'image|nullable|max:1999',
'objective' => 'required',
'company_name' => 'required'
]);
//Handle file upload
if($request->hasFile('admin_image')){
//Get file name with the extension
$fileNameWithExt = $request->file('admin_image')->getClientOriginalName();
//Get just filename
$filename = pathinfo($fileNameWithExt,PATHINFO_FILENAME);
//Get just extension
$extension = $request->file('admin_image')->getClientOriginalExtension();
//Filename to store
$fileNameToStore = $filename.'_'.time().'.'.$extension;
//Upload image
$path = $request->file('admin_image')->storeAs('public/admin_image',$fileNameToStore);
}else {
$fileNameToStore = 'noimage.jpg';
}
$admin = new AdminProfile;
$admin->address = $request->address;
$admin->user_id = auth()->user()->id;
$admin->user_name = auth()->user()->name;
$admin->user_email = auth()->user()->email;
$admin->user_usertype = auth()->user()->usertype;
$admin->phone = $request->phone;
$admin->no_of_staff = $request->no_of_staff;
$admin->admin_image = $fileNameToStore;
$admin->objective = $request->objective;
$admin->company_name = $request->company_name;
if($admin->save()){
notify()->success("Profile Created!","Success");
}
else{
notify()->error("There was a problem creating your profile!","Error");
}
return redirect('/dashboard');
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show($id)
{
$admin = AdminProfile::find($id);
//dd($admin);
return view('adminprofile.show')->with('admin',$admin);
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function edit($id)
{
$admin = AdminProfile::find($id);
//dd($admin->all());
return view('adminprofile.edit')->with('admin',$admin);
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id)
{
dd($request->all());
$this->validate($request,[
'address' => 'required',
'phone' => 'required',
'no_of_staff' => 'required',
'objective' => 'required',
'company_name' => 'required'
]);
if($request->hasFile('admin_image')){
//Get file name with the extension
$fileNameWithExt = $request->file('admin_image')->getClientOriginalName();
//Get just filename
$filename = pathinfo($fileNameWithExt,PATHINFO_FILENAME);
//Get just extension
$extension = $request->file('admin_image')->getClientOriginalExtension();
//Filename to store
$fileNameToStore = $filename.'_'.time().'.'.$extension;
//Upload image
$path = $request->file('admin_image')->storeAs('public/admin_image',$fileNameToStore);
}
$admin = AdminProfile::find($id);
$admin->address = $request->address;
$admin->phone = $request->phone;
$admin->no_of_staff = $request->no_of_staff;
$admin->objective = $request->objective;
$admin->company_name = $request->company_name;
if($request->hasFile('admin_image')){
$admin->admin_image = $fileNameToStore;
}
if($admin->save()){
notify()->success("Profile Updated!","Success");
}else{
notify()->error("There was a problem creating your profile!","Error");
}
return redirect()->back();
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id)
{
$admin_user = User::whereId($id);
//dd($admin_user);
/*if($admin_user->admin_image !== 'noimage.jpg'){
//Delete image
Storage::delete('public/admin_image/'.$admin_user->admin_image);
}*/
$admin_user->delete();
return redirect('/');
}
}
<file_sep>/app/Http/Controllers/TransactionsController.php
<?php
namespace App\Http\Controllers;
use App\Account;
use App\Transaction;
use Illuminate\Http\Request;
class TransactionsController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{ $account = Account::orderBy('id','desc')->get();
$transactions = Transaction::orderBy('id','asc')->get();
return view('transactions.index')->with('transactions',$transactions)
->with('account',$account);
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
$accounts = Account::orderBy('id','desc')->get();
//dd($account);
return view('transactions.create')->with('accounts',$accounts);
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
$this->validate($request,[
'transaction_type'=>'required',
'account_id'=>'required',
'transaction_with'=>'required',
'amount'=>'required'
]);
$transaction = new Transaction;
$transaction->transaction_type = $request->transaction_type;
$transaction->user_id = auth()->user()->id;
$transaction->account_id = $request->account_id;
$transaction->transaction_with = $request->transaction_with;
$transaction->amount = $request->amount;
$transaction->updateAccountBalance('create');
$transaction->save();
notify()->success("Transaction Created!","Success");
return redirect()->back();
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show($id)
{
//
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function edit($id)
{
$account = Account::orderBy('id','desc')->get();
$transaction = Transaction::findOrFail($id);
return view('transactions.edit')->with('account', $account)
->with('transaction',$transaction);
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id)
{
notify()->error("Transaction Can not be edited!","Error");
return redirect()->back();
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id)
{
$transaction = Transaction::findorfail($id);
$transaction->updateAccountBalance('delete');
$transaction->delete();
notify()->error("You have successfully deleted Transaction!","Error");
return redirect()->back();
}
}
<file_sep>/app/Http/Controllers/CustomersController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Storage;
use RealRashid\SweetAlert\Facades\Alert;
use App\Staffs;
use App\User;
use App\AdminProfile;
use DB;
use App\Customers;
class CustomersController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
$customers = Customers::orderBy('id','desc')->get();
return view('customers.index')->with('customers',$customers);
}
public function Customer_PDF()
{
$customer_pdf = Customers::orderBy('id','desc')->get();
$filename = 'Customers_list.pdf';
$mpdf = new \Mpdf\Mpdf([
'margin-left' => 10,
'margin-left' => 10,
'margin-left' => 15,
'margin-left' => 20,
'margin-left' => 10,
'margin-left' => 10
]);
$html = \View::make('customers.customerPDF')->with('customer_pdf',$customer_pdf);
$html = $html->render();
$mpdf->setHeader('xxxx|Customers|{PAGENO}');
$mpdf->setFooter('Copyright StockBase Inc');
$stylesheet = file_get_contents(url('css/customer_pdf.css'));
$mpdf->WriteHTML($stylesheet,1);
$mpdf->WriteHTML($html);
$mpdf->Output($filename,'I');
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
return view('customers.create');
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
$this->validate($request,[
'avatar'=>'image|nullable|max:1999',
'name'=>'max:185',
'email'=>'max:100',
'address'=>'max:185',
'city'=>'max:185',
'state'=>'max:185',
'company_name'=>'max:150',
'account'=>'max:150',
'zip'=>'max:10',
'phone_number'=>'max:20',
'prev_balance'=>'max:999999|numeric',
'payment'=>'max:999999|numeric|nullable'
]);
//Handle file uploads
if($request->hasFile('avatar')){
//Get file name with the extension
$fileNameWithExt = $request->file('avatar')->getClientOriginalName();
//Get just filename
$filename = pathinfo($fileNameWithExt,PATHINFO_FILENAME);
//Get just extension
$extension = $request->file('avatar')->getClientOriginalExtension();
//Filename to store
$fileNameToStore = $filename.'_'.time().'.'.$extension;
//Upload image
$path = $request->file('avatar')->storeAs('public/avatar',$fileNameToStore);
} else {
$fileNameToStore = 'noimage.jpg';
}
//dd($request->all());
$customer = new Customers;
$customer->name = $request->name;
$customer->email = $request->email;
$customer->phone_number = $request->phone_number;
$customer->avatar = $fileNameToStore;
$customer->address = $request->address;
$customer->city = $request->city;
$customer->state = $request->state;
$customer->zip = $request->zip;
$customer->company_name = $request->company_name;
$customer->account = $request->account;
$customer->prev_balance = $request->prev_balance;
$customer->payment = $request->payment;
if($customer->save()){
notify()->success("Customer Added!","Success");
}else{
notify()->error("There was a problem creating customer!","Error");
}
return redirect()->back();
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show($id)
{
$customer = Customers::find($id);
return view('customers.show')->with('customer',$customer);
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function edit($id)
{
$customer = Customers::findorfail($id);
return view('customers.edit')->with('customer',$customer);
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id)
{
$this->validate($request,[
'name'=>'max:185',
'email'=>'max:100',
'address'=>'max:185',
'city'=>'max:185',
'state'=>'max:185',
'company_name'=>'max:50',
'account'=>'max:50',
'zip'=>'max:10',
'phone_number'=>'max:20',
'prev_balance'=>'max:999999|numeric',
'payment'=>'max:999999|numeric|nullable'
]);
if($request->hasFile('avatar')){
//Get file name with the extension
$fileNameWithExt = $request->file('avatar')->getClientOriginalName();
//Get just filename
$filename = pathinfo($fileNameWithExt,PATHINFO_FILENAME);
//Get just extension
$extension = $request->file('avatar')->getClientOriginalExtension();
//Filename to store
$fileNameToStore = $filename.'_'.time().'.'.$extension;
//Upload image
$path = $request->file('avatar')->storeAs('public/avatar',$fileNameToStore);
}
//dd($request->all());
// dd("am here");
$customer = Customers::find($id);
$customer->name = $request->name;
$customer->email = $request->email;
$customer->phone_number = $request->phone_number;
$customer->address = $request->address;
$customer->city = $request->city;
$customer->state = $request->state;
$customer->zip = $request->zip;
$customer->company_name = $request->company_name;
$customer->account = $request->account;
$customer->prev_balance = $request->prev_balance;
$customer->payment = $request->payment;
if($request->hasFile('avatar')){
$customer->avatar = $fileNameToStore;
}
if($customer->save()){
notify()->success("Customer Updated!","Success");
}else{
notify()->error("There was a problem creating your profile!","Error");
}
return redirect()->route('customers.index');
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id)
{
$customer = Customers::findorfail($id);
$customer->delete();
notify()->success("Customer Deleted!","Success");
return redirect()->back();
}
}
<file_sep>/app/Http/Controllers/SaleReportController.php
<?php
namespace App\Http\Controllers;
use App\Sale;
use Illuminate\Http\Request;
class SaleReportController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
$salesReport = Sale::orderBy('id','desc')->paginate('10');
// dd($salesReport);
return view('report.sale')->with('saleReport', $salesReport);
}
public function getSales(Request $request)
{
if ($request->ajax()) {
$saleReport = Sale::whereBetween('created_at', [ $request->DateCreated.' 00:00:00', $request->EndDate.' 23:59:59'])->get();
return view('report.listssale')->with('saleReport', $saleReport);
}
}
public function Sale_PDF()
{
$sales_pdf = Sale::orderBy('id','desc')->get();
$filename = 'sales_report.pdf';
$mpdf = new \Mpdf\Mpdf([
'margin-left' => 10,
'margin-left' => 10,
'margin-left' => 15,
'margin-left' => 20,
'margin-left' => 10,
'margin-left' => 10
]);
$html = \View::make('sales.salesreportPDF')->with('sales_pdf',$sales_pdf);
$html = $html->render();
$mpdf->setHeader('xxxx|Sales-Report|{PAGENO}');
$mpdf->setFooter('Copyright StockBase Inc');
$stylesheet = file_get_contents(url('css/customer_pdf.css'));
$mpdf->WriteHTML($stylesheet,1);
$mpdf->WriteHTML($html);
$mpdf->Output($filename,'I');
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
//
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
//
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show($id)
{
//
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function edit($id)
{
//
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id)
{
//
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id)
{
//
}
}
| dd9bd40c3e0fc1139399431e122f533df46482cf | [
"JavaScript",
"Markdown",
"PHP"
] | 26 | PHP | ayodvr/Laravel-POS-application | 8b231d798cc5d5bd6e0d313e527bdbff2bf2a67c | be2e9efb94130840fffd6d7b593a2ad4e7efe2e5 | |
refs/heads/master | <file_sep>package io.github.devas;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.Scanner;
class MessengerApp {
private boolean isRunning = true;
private Sender sender;
private Receiver receiver;
private Configuration conf = new Configuration(7777, "Anakin", "localhost", 8888);
void startMessenger() throws IOException {
Scanner sc = new Scanner(System.in);
System.out.print("Listening port: ");
conf.setListeningPort(sc.nextInt());
receiver = new Receiver(conf.getListeningPort());
System.out.print("Target port: ");
conf.setTargetPort(sc.nextInt());
sender = new Sender(conf.getUserName(), conf.getTargetIp(), conf.getTargetPort());
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
System.out.println("Chat started. Type '\\exit' to quit.");
while (isRunning) {
String input = br.readLine();
if (input.equals("\\exit")) {
receiver.stop();
sender.stop();
isRunning = false;
} else {
sender.sendMessage(input);
}
}
}
}
<file_sep>package io.github.devas;
class Configuration {
private int listeningPort;
private String userName;
private String targetIp;
private int targetPort;
Configuration(int listeningPort, String userName, String targetIp, int targetPort) {
this.listeningPort = listeningPort;
this.userName = userName;
this.targetIp = targetIp;
this.targetPort = targetPort;
}
int getListeningPort() {
return listeningPort;
}
void setListeningPort(int listeningPort) {
this.listeningPort = listeningPort;
}
String getUserName() {
return userName;
}
void setUserName(String userName) {
this.userName = userName;
}
String getTargetIp() {
return targetIp;
}
void setTargetIp(String targetIp) {
this.targetIp = targetIp;
}
int getTargetPort() {
return targetPort;
}
void setTargetPort(int targetPort) {
this.targetPort = targetPort;
}
}
| fa812c0bd4e73eff9345af7435c395708569d4a8 | [
"Java"
] | 2 | Java | Devas/Messenger | 6b1aa6dba5d00fa27e0e2b30f27ae6754dd1c9e6 | 82b59133d83478d1f8286d63531338ddfae5a7e5 | |
refs/heads/master | <repo_name>mama-byte/rails-longest-word-game<file_sep>/app/controllers/games_controller.rb
require 'json'
require 'open-uri'
class GamesController < ApplicationController
def new
@time = Time.new
@letters = []
('a'..'z').to_a.sample(10).each do |letter|
@letters << letter
end
@letters
end
def score
@input = params[:word]
parsed_json(@input)
@time_taken = Time.new - Time.parse(params[:time])
end
def parsed_json(attempt)
url = "http://wagon-dictionary.herokuapp.com/#{attempt}"
words = open(url).read
word = JSON.parse(words)
end
end
| 7dc6da62a3e8cd2911b0c88e2031614502c8a02e | [
"Ruby"
] | 1 | Ruby | mama-byte/rails-longest-word-game | d0b839c42c40b5cac4d29e1c133cbfa3b0e92b74 | 882558a2a1c70955fcd04dc30847625000fbecce | |
refs/heads/master | <repo_name>rcsarv/rockmongo-docker<file_sep>/www/default/vendor/alcaeus/mongo-php-adapter/tests/Alcaeus/MongoDbAdapter/Mongo/MongoClientTest.php
<?php
namespace Alcaeus\MongoDbAdapter\Tests\Mongo;
use Alcaeus\MongoDbAdapter\Tests\TestCase;
/**
* @author alcaeus <<EMAIL>>
*/
class MongoClientTest extends TestCase
{
public function testSerialize()
{
$this->assertInternalType('string', serialize($this->getClient()));
}
public function testGetDb()
{
$client = $this->getClient();
$db = $client->selectDB('mongo-php-adapter');
$this->assertInstanceOf('\MongoDB', $db);
$this->assertSame('mongo-php-adapter', (string) $db);
}
public function testSelectDBWithEmptyName()
{
$this->setExpectedException('Exception', 'Database name cannot be empty');
$this->getClient()->selectDB('');
}
public function testSelectDBWithInvalidName()
{
$this->setExpectedException('Exception', 'Database name contains invalid characters');
$this->getClient()->selectDB('/');
}
public function testGetDbProperty()
{
$client = $this->getClient();
$db = $client->{'mongo-php-adapter'};
$this->assertInstanceOf('\MongoDB', $db);
$this->assertSame('mongo-php-adapter', (string) $db);
}
public function testGetCollection()
{
$client = $this->getClient();
$collection = $client->selectCollection('mongo-php-adapter', 'test');
$this->assertInstanceOf('MongoCollection', $collection);
$this->assertSame('mongo-php-adapter.test', (string) $collection);
}
public function testGetHosts()
{
$client = $this->getClient();
$hosts = $client->getHosts();
$this->assertArraySubset(
[
'localhost:27017;-;.;' . getmypid() => [
'host' => 'localhost',
'port' => 27017,
'health' => 1,
'state' => 0,
],
],
$hosts
);
}
public function testReadPreference()
{
$client = $this->getClient();
$this->assertSame(['type' => \MongoClient::RP_PRIMARY], $client->getReadPreference());
$this->assertTrue($client->setReadPreference(\MongoClient::RP_SECONDARY, [['a' => 'b']]));
$this->assertSame(['type' => \MongoClient::RP_SECONDARY, 'tagsets' => [['a' => 'b']]], $client->getReadPreference());
}
public function testWriteConcern()
{
$client = $this->getClient();
$this->assertTrue($client->setWriteConcern('majority', 100));
$this->assertSame(['w' => 'majority', 'wtimeout' => 100], $client->getWriteConcern());
}
public function testListDBs()
{
$document = ['foo' => 'bar'];
$this->getCollection()->insert($document);
$databases = $this->getClient()->listDBs();
$this->assertSame(1.0, $databases['ok']);
$this->assertArrayHasKey('totalSize', $databases);
$this->assertArrayHasKey('databases', $databases);
foreach ($databases['databases'] as $database) {
$this->assertArrayHasKey('name', $database);
$this->assertArrayHasKey('empty', $database);
$this->assertArrayHasKey('sizeOnDisk', $database);
if ($database['name'] == 'mongo-php-adapter') {
$this->assertFalse($database['empty']);
return;
}
}
$this->fail('Could not find mongo-php-adapter database in list');
}
public function testNoPrefixUri()
{
$client = $this->getClient(null, 'localhost');
$this->assertNotNull($client);
}
}
<file_sep>/www/default/logs/README.txt
Query logs goes here, you should make this directory writeable.
| cd1a0a956b9a73f9ee252c46bcbc58d048fbc7c5 | [
"Text",
"PHP"
] | 2 | PHP | rcsarv/rockmongo-docker | 95bb64792b819f06c5ad1a618ddb6da03743e7c7 | b2cc4065a159aaf50998d2c292a7774be52145f6 | |
refs/heads/main | <file_sep># NativeScript JavaScript Template
This template creates a "Hello, world" NativeScript app using JavaScript.
This is the default template, so you can create a new app that uses it with the `--template` option:
```
ns create my-hello-world-js --template @nativescript/template-hello-world
```
Or without it:
```
ns create my-hello-world-js
```
> Note: Both commands will create a new NativeScript app that uses the latest version of this template published to [npm](https://www.npmjs.com/package/@nativescript/template-hello-world).
If you want to create a new app that uses the source of the template from the `master` branch, you can execute the following:
```
# clone nativescript-app-templates monorepo locally
git clone <EMAIL>:NativeScript/nativescript-app-templates.git
# create app template from local source (all templates are in the 'packages' subfolder of the monorepo)
ns create my-hello-world-js --template nativescript-app-templates/packages/template-hello-world
```
## Get Help
The NativeScript framework has a vibrant community that can help when you run into problems.
Try [joining the NativeScript community Discord](https://nativescript.org/discord). The Discord channel is a great place to get help troubleshooting problems, as well as connect with other NativeScript developers.
If you have found an issue with this template, please report the problem in the [NativeScript repository](https://github.com/NativeScript/NativeScript/issues).
## Contributing
We love PRs, and accept them gladly. Feel free to propose changes and new ideas. We will review and discuss, so that they can be accepted and better integrated.
<file_sep>declare module '*.svelte' {
export { SvelteComponent as default };
}<file_sep>import Vue from 'nativescript-vue'
import App from './components/App'
new Vue({
render: (h) => h(App),
}).$start()
<file_sep>import { Observable } from '@nativescript/core'
export class HomeViewModel extends Observable {
constructor() {
super()
}
}
<file_sep>import { Component, OnInit } from '@angular/core'
import { NavigationEnd, Router } from '@angular/router'
import { RouterExtensions } from '@nativescript/angular'
import {
DrawerTransitionBase,
RadSideDrawer,
SlideInOnTopTransition,
} from 'nativescript-ui-sidedrawer'
import { filter } from 'rxjs/operators'
import { Application } from '@nativescript/core'
@Component({
selector: 'ns-app',
templateUrl: 'app.component.html',
})
export class AppComponent implements OnInit {
private _activatedUrl: string
private _sideDrawerTransition: DrawerTransitionBase
constructor(private router: Router, private routerExtensions: RouterExtensions) {
// Use the component constructor to inject services.
}
ngOnInit(): void {
this._activatedUrl = '/home'
this._sideDrawerTransition = new SlideInOnTopTransition()
this.router.events
.pipe(filter((event: any) => event instanceof NavigationEnd))
.subscribe((event: NavigationEnd) => (this._activatedUrl = event.urlAfterRedirects))
}
get sideDrawerTransition(): DrawerTransitionBase {
return this._sideDrawerTransition
}
isComponentSelected(url: string): boolean {
return this._activatedUrl === url
}
onNavItemTap(navItemRoute: string): void {
this.routerExtensions.navigate([navItemRoute], {
transition: {
name: 'fade',
},
})
const sideDrawer = <RadSideDrawer>Application.getRootView()
sideDrawer.closeDrawer()
}
}
<file_sep>import { EventData, View, NavigatedData, Page } from '@nativescript/core'
import { Item } from '../shared/item'
export function onNavigatingTo(args: NavigatedData) {
const page = args.object as Page
const item = args.context as Item
page.bindingContext = item
}
export function onBackButtonTap(args: EventData) {
const view = args.object as View
const page = view.page as Page
page.frame.goBack()
}
<file_sep># React NativeScript Blank Template
App templates help you jump start your native cross-platform apps with built-in UI elements and best practices. Save time writing boilerplate code over and over again when you create new apps.
## Quick Start from NPM package
Execute the following command to create an app from this template:
```
ns create my-blank-react --react
cd my-blank-react
npm install
ns preview
# or
ns run android
# or
ns run ios
```
> Note: This command will create a new NativeScript app that uses the latest version of this template published to [npm](https://www.npmjs.com/package/@nativescript/template-blank-react).
**NB:** Please, keep in mind that the master branch may refer to dependencies that are not on NPM yet!
## Walkthrough
Having created an app from this template, here's an introduction to how it works:
### Architecture
- `src/app.ts`: The app entrypoint. It renders the MainStack component as the root component.
- `src/components/MainStack.tsx`: A React Navigation 5 [stack navigator](https://reactnavigation.org/docs/5.x/stack-navigator/) serving as the root component.
- `src/components/ScreenOne.tsx`, `src/components/ScreenTwo.tsx`: A couple of screens to navigate to.
- `src/NavigationParamList.tsx`: A record of the navigation params for each route in your app.
### The basics
Learn React NativeScript itself by reading [its docs](https://react-nativescript.netlify.app/docs/introduction/introduction.html).
Learn how to navigate by referring to the React Navigation 5 [docs])https://reactnavigation.org/docs/5.x/getting-started) and refer to the [react-nativescript-navigation](https://github.com/shirakaba/react-nativescript-navigation/tree/master/react-nativescript-navigation) repo to see which options are supported for each navigator.
React libraries without a dependency on `react-dom` (like Redux) should work just fine in React NativeScript as long as the `react` version is compatible.
## Get Help
The NativeScript framework has a vibrant community that can help when you run into problems.
Try [joining the NativeScript community Discord](https://nativescript.org/discord). The Discord `#react` channel is a great place to get help troubleshooting problems, as well as connect with other NativeScript developers.
If you have found an issue with this template, please report the problem in this repository's [Issues page](https://github.com/shirakaba/tns-template-blank-react/issues).
## Contributing
We love PRs, and accept them gladly. Feel free to propose changes and new ideas. We will review and discuss, so that they can be accepted and better integrated.
<file_sep>export const carClassList: Array<string> = ['Mini', 'Economy', 'Compact', 'Standard', 'Luxury']
export const carDoorList: Array<number> = [2, 3, 5]
export const carSeatList: Array<string> = ['2', '4', '4 + 1', '6 + 1']
export const carTransmissionList: Array<string> = ['Manual', 'Automatic']
<file_sep>import { Application } from '@nativescript/core'
import { HomeViewModel } from './home-view-model'
export function onNavigatingTo(args) {
const page = args.object
page.bindingContext = new HomeViewModel()
}
export function onDrawerButtonTap(args) {
const sideDrawer = Application.getRootView()
sideDrawer.showDrawer()
}
<file_sep>export const classList = ['Mini', 'Economy', 'Compact', 'Standard', 'Luxury']
export const doorsList = [2, 3, 5]
export const seatsList = ['2', '4', '4 + 1', '6 + 1']
export const transmissionList = ['Manual', 'Automatic']
<file_sep>import { NgModule, NO_ERRORS_SCHEMA } from '@angular/core'
import { NativeScriptCommonModule } from '@nativescript/angular'
import { FeaturedRoutingModule } from './featured-routing.module'
import { FeaturedComponent } from './featured.component'
@NgModule({
imports: [NativeScriptCommonModule, FeaturedRoutingModule],
declarations: [FeaturedComponent],
schemas: [NO_ERRORS_SCHEMA],
})
export class FeaturedModule {}
<file_sep>export function Car(options) {
const model = {
id: options.id,
name: options.name,
hasAC: options.hasAC,
description: options.description,
seats: options.seats,
luggage: Number(options.luggage),
class: options.class,
doors: Number(options.doors),
price: Number(options.price),
transmission: options.transmission,
imageUrl: options.imageUrl,
imageStoragePath: options.imageStoragePath,
}
return model
}
<file_sep>import db from "./car-rental-export-public.json"
class ApiService {
constructor() {
this.db = db;
}
update(path, updateModel) {
const carId = path.split("/").slice(-1)[0];
this.db.cars[carId] = {...this.db.cars[carId], ...updateModel }
}
uploadFile(params) {
return {url: params.localFullPath}
}
addValueEventListener(onValueEvent, path) {
onValueEvent(this.db.cars)
return {
path: path,
listeners: this.db.cars
}
}
}
export default new ApiService;
<file_sep>export const visibilityValueConverter = {
toView: function (value) {
if (value) {
return 'collapsed'
} else {
return 'visible'
}
},
toModel: function (value) {
return value
},
}
<file_sep>import { Subscription } from 'rxjs'
import { finalize } from 'rxjs/operators'
import { Observable, ObservableArray } from '@nativescript/core'
import { ObservableProperty } from '../shared/observable-property-decorator'
import { Car } from './shared/car-model'
import { CarService } from './shared/car-service'
export class CarsListViewModel extends Observable {
private static _subscriptionKey = 'car-list-view-model'
@ObservableProperty() cars: ObservableArray<Car>
@ObservableProperty() isLoading: boolean
private _carService: CarService
private _dataSubscription: Subscription
constructor() {
super()
this.cars = new ObservableArray<Car>([])
this.isLoading = false
this._carService = CarService.getInstance()
}
load(): void {
if (!this._dataSubscription) {
this.isLoading = true
// we need to be able to unsubscribe from data changes when old page got disposed / inaccessible but
// we do not have equivalent of Angular ngOnInit / ngOnDestroy so it cannot be implicit solution i.e.
// we should explicitly state when to unsubscribe e.g. in the following scenario master list #1 ->
// car detail -> edit car detail -> master list #2 (forward nav with clearHistory)
// we need to unsubscribe the master list #1 view model (as it can never be accessed again)
// if cached subscription exists here, we know it is from a different (previous) instance of the same
// view model and we need to unsubscribe from it
const cachedSubscription = this._carService.getSubscription(
CarsListViewModel._subscriptionKey
)
if (cachedSubscription) {
cachedSubscription.unsubscribe()
this._carService.setSubscription(CarsListViewModel._subscriptionKey, null)
}
this._dataSubscription = this._carService
.load()
.pipe(
finalize(() => {
this.isLoading = false
})
)
.subscribe((cars: Array<Car>) => {
this.cars = new ObservableArray(cars)
this.isLoading = false
})
this._carService.setSubscription(CarsListViewModel._subscriptionKey, this._dataSubscription)
}
}
}
<file_sep>import { HomeItemsViewModel } from './home-items-view-model'
export function onNavigatingTo(args) {
const component = args.object
component.bindingContext = new HomeItemsViewModel()
}
export function onItemTap(args) {
const view = args.view
const page = view.page
const tappedItem = view.bindingContext
page.frame.navigate({
moduleName: 'home/home-item-detail/home-item-detail-page',
context: tappedItem,
animated: true,
transition: {
name: 'slide',
duration: 200,
curve: 'ease',
},
})
}
<file_sep>import { Application } from '@nativescript/core'
import { SearchViewModel } from './search-view-model'
export function onNavigatingTo(args) {
const page = args.object
page.bindingContext = new SearchViewModel()
}
export function onDrawerButtonTap(args) {
const sideDrawer = Application.getRootView()
sideDrawer.showDrawer()
}
<file_sep>import Vue from 'nativescript-vue'
import RadListView from 'nativescript-ui-listview/vue'
Vue.use(RadListView)
import App from './components/App'
Vue.config.silent = !__DEV__
new Vue({
render: h => h(App)
}).$start()
<file_sep>import { platformNativeScript, runNativeScriptAngularApp } from '@nativescript/angular';
import { AppModule } from './app/app.module';
runNativeScriptAngularApp({
appModuleBootstrap: () => platformNativeScript().bootstrapModule(AppModule),
});
<file_sep>import { Component, EventEmitter, Input, OnInit, Output, ViewContainerRef } from '@angular/core'
import { ModalDialogOptions, ModalDialogService, PageRoute } from '@nativescript/angular'
import { switchMap } from 'rxjs/operators'
import { CarEditService } from '../../shared/car-edit.service'
import { Car } from '../../shared/car.model'
import { MyListSelectorModalViewComponent } from './my-list-selector-modal-view.component'
const capitalizeFirstLetter = (s) => s.charAt(0).toUpperCase() + s.slice(1)
@Component({
providers: [ModalDialogService],
selector: 'MyListSelector',
templateUrl: './my-list-selector.component.html',
})
export class MyListSelectorComponent implements OnInit {
@Input() tag: string
@Input() items: Array<string>
@Input() selectedValue: string
@Output() selectedValueChange = new EventEmitter<string>()
private _carEditModel: Car
constructor(
private _pageRoute: PageRoute,
private _modalService: ModalDialogService,
private _vcRef: ViewContainerRef,
private _carEditService: CarEditService
) {}
ngOnInit(): void {
let carId = ''
this._pageRoute.activatedRoute
.pipe(switchMap((activatedRoute) => activatedRoute.params))
.forEach((params) => {
carId = params.id
})
this._carEditModel = this._carEditService.getEditableCarById(carId)
}
onSelectorTap(): void {
const title = `Select Car ${capitalizeFirstLetter(this.tag)}`
const selectedIndex = this.items.indexOf(this.selectedValue)
const options: ModalDialogOptions = {
viewContainerRef: this._vcRef,
context: {
items: this.items,
title,
selectedIndex,
},
fullscreen: false,
}
this._modalService
.showModal(MyListSelectorModalViewComponent, options)
.then((selectedValue: string) => {
if (selectedValue) {
this.selectedValue = selectedValue
this.selectedValueChange.emit(this.selectedValue)
}
})
}
}
<file_sep>import { Frame } from '@nativescript/core'
import { CarDetailViewModel } from './car-detail-view-model'
export function onNavigatingTo(args) {
if (args.isBackNavigation) {
return
}
const page = args.object
page.bindingContext = new CarDetailViewModel(page.navigationContext)
}
export function onBackButtonTap() {
Frame.topmost().goBack()
}
export function onEditButtonTap(args) {
const bindingContext = args.object.bindingContext
Frame.topmost().navigate({
moduleName: 'cars/car-detail-edit-page/car-detail-edit-page',
context: bindingContext.car,
animated: true,
transition: {
name: 'slideTop',
duration: 200,
curve: 'ease',
},
})
}
<file_sep>import { Observable } from '@nativescript/core'
import { carClassList, carDoorList, carSeatList, carTransmissionList } from './constants'
export class ListSelectorViewModel extends Observable {
private _items: Array<any>
private _tag: string
private _selectedIndex: number
constructor(context: any, private _closeCallback: Function) {
super()
this._tag = context.tag
const protoItems = this.resolveProtoItems()
this._selectedIndex = protoItems.indexOf(context.selectedValue)
this._items = []
for (let i = 0; i < protoItems.length; i++) {
this._items.push({
value: protoItems[i],
isSelected: i === this._selectedIndex ? true : false,
})
}
}
selectItem(newSelectedIndex: number): void {
const oldSelectedItem = this._items[this._selectedIndex]
oldSelectedItem.isSelected = false
const newSelectedItem = this._items[newSelectedIndex]
newSelectedItem.isSelected = true
this._selectedIndex = newSelectedIndex
this._closeCallback(newSelectedItem.value)
}
cancelSelection(): void {
this._closeCallback(null)
}
get items(): Array<any> {
return this._items
}
get title(): string {
return `Select Car ${this.capitalizeFirstLetter(this._tag)}`
}
private resolveProtoItems(): Array<any> {
switch (this._tag) {
case 'class':
return carClassList
case 'doors':
return carDoorList
case 'seats':
return carSeatList
case 'transmission':
return carTransmissionList
}
}
private capitalizeFirstLetter(str: string): string {
return str.charAt(0).toUpperCase() + str.slice(1)
}
}
<file_sep>import { ShownModallyData, View } from '@nativescript/core'
import { ListSelectorViewModel } from './list-selector-view-model'
let viewModel: ListSelectorViewModel
export function onShownModally(args: ShownModallyData): void {
viewModel = new ListSelectorViewModel(args.context, args.closeCallback)
;(<View>args.object).bindingContext = viewModel
}
export function onItemSelected(args): void {
viewModel.selectItem(args.index)
}
export function onCancelButtonTap(): void {
viewModel.cancelSelection()
}
<file_sep>import { catchError } from 'rxjs/operators'
import { Observable, throwError } from 'rxjs'
import { Car } from './car-model'
import ApiService from "~/services/api.service";
const editableProperties = [
'doors',
'imageUrl',
'luggage',
'name',
'price',
'seats',
'transmission',
'class',
]
export function CarService() {
if (CarService._instance) {
throw new Error('Use CarService.getInstance() instead of new.')
}
this._cars = []
this._subscriptionMap = new Map()
CarService._instance = this
this.getSubscription = function (key) {
return this._subscriptionMap.get(key)
}
this.setSubscription = function (key, value) {
this._subscriptionMap.set(key, value)
}
this.load = function () {
return new Observable((observer) => {
const path = 'cars'
const onValueEvent = (snapshot) => {
const results = this._handleSnapshot(snapshot)
observer.next(results)
}
ApiService.addValueEventListener(onValueEvent, `/${path}`)
}).pipe(catchError(this._handleErrors))
}
this.update = function (carModel) {
const updateModel = cloneUpdateModel(carModel)
return ApiService.update(`/cars/${carModel.id}`, updateModel)
}
this.uploadImage = function (remoteFullPath, localFullPath) {
return ApiService.uploadFile({
localFullPath,
remoteFullPath,
onProgress: null,
})
}
this._handleSnapshot = function (data) {
this._cars = []
if (data) {
for (const id in data) {
if (data.hasOwnProperty(id)) {
this._cars.push(new Car(data[id]))
}
}
}
return this._cars
}
this._handleErrors = function (error) {
return throwError(error)
}
}
CarService.getInstance = function () {
return CarService._instance
}
CarService._instance = new CarService()
function cloneUpdateModel(car) {
return editableProperties.reduce((a, e) => ((a[e] = car[e]), a), {}) // eslint-disable-line no-return-assign, no-sequences
}
<file_sep>import { Observable } from '@nativescript/core'
import { ObservableProperty } from '../../shared/observable-property-decorator'
export class Car extends Observable {
@ObservableProperty() id: string
@ObservableProperty() imageStoragePath: string
@ObservableProperty() seats: string
@ObservableProperty() luggage: number
@ObservableProperty() class: string
@ObservableProperty() hasAC: boolean
@ObservableProperty() doors: number
@ObservableProperty() price: number
@ObservableProperty() transmission: string
@ObservableProperty() isModelValid: boolean
private _name: string
private _imageUrl: string
constructor(options: any) {
super()
this._name = options.name
this._imageUrl = options.imageUrl
this.id = options.id
this.seats = options.seats
this.luggage = Number(options.luggage)
this.class = options.class
this.hasAC = options.hasAC
this.doors = Number(options.doors)
this.price = Number(options.price)
this.transmission = options.transmission
this.imageStoragePath = options.imageStoragePath
this.updateDependentProperties()
}
get name(): string {
return this._name
}
set name(value: string) {
if (this._name === value) {
return
}
this._name = value
this.notifyPropertyChange('name', value)
this.updateDependentProperties()
}
get imageUrl(): string {
return this._imageUrl
}
set imageUrl(value: string) {
if (this._imageUrl === value) {
return
}
this._imageUrl = value
this.notifyPropertyChange('imageUrl', value)
this.updateDependentProperties()
}
private updateDependentProperties(): void {
this.isModelValid = !!this._name && !!this._imageUrl
}
}
<file_sep>import Vue from 'nativescript-vue'
import Home from './components/Home'
new Vue({
render: (h) => h('frame', [h(Home)]),
}).$start()
<file_sep>import { Observable } from '@nativescript/core'
import { Car } from '../shared/car-model'
export class CarDetailViewModel extends Observable {
constructor(private _car: Car) {
super()
}
get car(): Car {
return this._car
}
}
<file_sep>import { Frame, NavigatedData, Page } from '@nativescript/core'
import { CarDetailViewModel } from './car-detail-view-model'
export function onNavigatingTo(args: NavigatedData): void {
if (args.isBackNavigation) {
return
}
const page = <Page>args.object
page.bindingContext = new CarDetailViewModel(page.navigationContext)
}
export function onBackButtonTap(): void {
Frame.topmost().goBack()
}
export function onEditButtonTap(args): void {
const bindingContext = <CarDetailViewModel>args.object.bindingContext
Frame.topmost().navigate({
moduleName: 'cars/car-detail-edit-page/car-detail-edit-page',
context: bindingContext.car,
animated: true,
transition: {
name: 'slideTop',
duration: 200,
curve: 'ease',
},
})
}
<file_sep>import { Component, OnDestroy, OnInit } from '@angular/core'
import { RouterExtensions } from '@nativescript/angular'
import { ListViewEventData } from 'nativescript-ui-listview'
import { Subscription } from 'rxjs'
import { finalize } from 'rxjs/operators'
import { ObservableArray } from '@nativescript/core'
import { Car } from './shared/car.model'
import { CarService } from './shared/car.service'
@Component({
selector: 'CarsList',
templateUrl: './car-list.component.html',
styleUrls: ['./car-list.component.scss'],
})
export class CarListComponent implements OnInit, OnDestroy {
private _isLoading: boolean = false
private _cars: ObservableArray<Car> = new ObservableArray<Car>([])
private _dataSubscription: Subscription
constructor(private _carService: CarService, private _routerExtensions: RouterExtensions) {}
ngOnInit(): void {
if (!this._dataSubscription) {
this._isLoading = true
this._dataSubscription = this._carService
.load()
.pipe(finalize(() => (this._isLoading = false)))
.subscribe((cars: Array<Car>) => {
this._cars = new ObservableArray(cars)
this._isLoading = false
})
}
}
ngOnDestroy(): void {
if (this._dataSubscription) {
this._dataSubscription.unsubscribe()
this._dataSubscription = null
}
}
get cars(): ObservableArray<Car> {
return this._cars
}
get isLoading(): boolean {
return this._isLoading
}
onCarItemTap(args: ListViewEventData): void {
const tappedCarItem = args.view.bindingContext
this._routerExtensions.navigate(['/cars/car-detail', tappedCarItem.id], {
animated: true,
transition: {
name: 'slide',
duration: 200,
curve: 'ease',
},
})
}
}
<file_sep>import { Observable } from '@nativescript/core'
export class HelloWorldModel extends Observable {
private _counter: number
private _message: string
constructor() {
super()
// Initialize default values.
this._counter = 42
this.updateMessage()
}
get message(): string {
return this._message
}
set message(value: string) {
if (this._message !== value) {
this._message = value
this.notifyPropertyChange('message', value)
}
}
onTap() {
this._counter--
this.updateMessage()
}
private updateMessage() {
if (this._counter <= 0) {
this.message = 'Hoorraaay! You unlocked the NativeScript clicker achievement!'
} else {
this.message = `${this._counter} taps left`
}
}
}
<file_sep>import { Frame, Application } from '@nativescript/core';
import { AppRootViewModel } from './app-root-view-model'
export function onLoaded(args) {
const drawerComponent = args.object
drawerComponent.bindingContext = new AppRootViewModel()
}
export function onNavigationItemTap(args) {
const component = args.object
const componentRoute = component.route
const componentTitle = component.title
const bindingContext = component.bindingContext
bindingContext.set('selectedPage', componentTitle)
Frame.topmost().navigate({
moduleName: componentRoute,
transition: {
name: 'fade',
},
})
const drawerComponent = Application.getRootView()
drawerComponent.closeDrawer()
}
<file_sep>import { Application } from '@nativescript/core'
export const showDrawer = () => {
let drawerNativeView = Application.getRootView()
if (drawerNativeView && drawerNativeView.showDrawer) {
drawerNativeView.showDrawer()
}
}
export const closeDrawer = () => {
let drawerNativeView = Application.getRootView()
if (drawerNativeView && drawerNativeView.showDrawer) {
drawerNativeView.closeDrawer()
}
}
<file_sep>import * as imagePicker from '@nativescript/imagepicker'
import { Observable, Folder, knownFolders, path, ImageAsset, ImageSource } from '@nativescript/core'
import { ObservableProperty } from '../../shared/observable-property-decorator'
import { Car } from '../shared/car-model'
import { CarService } from '../shared/car-service'
import { RoundingValueConverter } from './roundingValueConverter'
import { VisibilityValueConverter } from './visibilityValueConverter'
const tempImageFolderName = 'nsimagepicker'
export class CarDetailEditViewModel extends Observable {
static get imageTempFolder(): Folder {
return knownFolders.temp().getFolder(tempImageFolderName)
}
private static clearImageTempFolder(): void {
CarDetailEditViewModel.imageTempFolder.clear()
}
@ObservableProperty() car: Car
@ObservableProperty() isUpdating: boolean
private _roundingValueConverter: RoundingValueConverter
private _visibilityValueConverter: VisibilityValueConverter
private _isCarImageDirty: boolean
private _carService: CarService
constructor(car: Car) {
super()
// get a fresh editable copy of car model
this.car = new Car(car)
this.isUpdating = false
this._carService = CarService.getInstance()
this._isCarImageDirty = false
// set up value converter to force iOS UISlider to work with discrete steps
this._roundingValueConverter = new RoundingValueConverter()
this._visibilityValueConverter = new VisibilityValueConverter()
}
get roundingValueConverter(): RoundingValueConverter {
return this._roundingValueConverter
}
get visibilityValueConverter(): VisibilityValueConverter {
return this._visibilityValueConverter
}
saveChanges(): Promise<any> {
let queue = Promise.resolve()
this.isUpdating = true
// TODO: car image should be required field
if (this._isCarImageDirty && this.car.imageUrl) {
queue = queue
.then(() => {
// no need to explicitly delete old image as upload to an existing remote path overwrites it
const localFullPath = this.car.imageUrl
const remoteFullPath = this.car.imageStoragePath
return this._carService.uploadImage(remoteFullPath, localFullPath)
})
.then((uploadedFile: any) => {
this.car.imageUrl = uploadedFile.url
this._isCarImageDirty = false
})
}
return queue
.then(() => {
return this._carService.update(this.car)
})
.then(() => (this.isUpdating = false))
.catch((errorMessage: any) => {
this.isUpdating = false
throw errorMessage
})
}
onImageAddRemove(): void {
if (this.car.imageUrl) {
this.handleImageChange(null)
return
}
CarDetailEditViewModel.clearImageTempFolder()
this.pickImage()
}
private pickImage(): void {
const context = imagePicker.create({
mode: 'single',
})
context
.authorize()
.then(() => context.present())
.then((selection) =>
selection.forEach((selectedAsset: ImageAsset) => {
selectedAsset.options.height = 768
ImageSource.fromAsset(selectedAsset).then((imageSource: ImageSource) =>
this.handleImageChange(imageSource)
)
})
)
.catch((errorMessage: any) => console.log(errorMessage))
}
private handleImageChange(source: ImageSource): void {
let raisePropertyChange = true
let tempImagePath = null
if (source) {
tempImagePath = path.join(CarDetailEditViewModel.imageTempFolder.path, `${Date.now()}.jpg`)
raisePropertyChange = source.saveToFile(tempImagePath, 'jpeg')
}
if (raisePropertyChange) {
this.car.imageUrl = tempImagePath
this._isCarImageDirty = true
}
}
}
<file_sep>import { ListViewEventData } from 'nativescript-ui-listview'
import { Frame, NavigatedData, Page } from '@nativescript/core'
import { CarsListViewModel } from './cars-list-view-model'
import { Car } from './shared/car-model'
export function onNavigatingTo(args: NavigatedData): void {
if (args.isBackNavigation) {
return
}
const viewModel = new CarsListViewModel()
viewModel.load()
const page = <Page>args.object
page.bindingContext = viewModel
}
export function onCarItemTap(args: ListViewEventData): void {
const tappedCarItem = <Car>args.view.bindingContext
Frame.topmost().navigate({
moduleName: 'cars/car-detail-page/car-detail-page',
context: tappedCarItem,
animated: true,
transition: {
name: 'slide',
duration: 200,
curve: 'ease',
},
})
}
<file_sep>import { Application } from '@nativescript/core'
Application.run({ moduleName: 'app-root' })
/*
Do not place any code after the application has been started as it will not
be executed on iOS.
*/
<file_sep>import Vue from 'nativescript-vue'
import RadSideDrawer from 'nativescript-ui-sidedrawer/vue'
Vue.use(RadSideDrawer)
import App from './components/App'
Vue.config.silent = !__DEV__
new Vue({
render: h => h(App)
}).$start()
<file_sep>import { Observable } from '@nativescript/core'
import { Item } from './shared/item'
export class HomeViewModel extends Observable {
items: Array<Item>
constructor() {
super()
this.items = new Array<Item>(
{
name: 'Item 1',
description: 'Description for Item 1',
},
{
name: 'Item 2',
description: 'Description for Item 2',
},
{
name: 'Item 3',
description: 'Description for Item 3',
},
{
name: 'Item 4',
description: 'Description for Item 4',
},
{
name: 'Item 5',
description: 'Description for Item 5',
},
{
name: 'Item 6',
description: 'Description for Item 6',
},
{
name: 'Item 7',
description: 'Description for Item 7',
},
{
name: 'Item 8',
description: 'Description for Item 8',
},
{
name: 'Item 9',
description: 'Description for Item 9',
},
{
name: 'Item 10',
description: 'Description for Item 10',
},
{
name: 'Item 11',
description: 'Description for Item 11',
},
{
name: 'Item 12',
description: 'Description for Item 12',
},
{
name: 'Item 13',
description: 'Description for Item 13',
},
{
name: 'Item 14',
description: 'Description for Item 14',
},
{
name: 'Item 15',
description: 'Description for Item 15',
},
{
name: 'Item 16',
description: 'Description for Item 16',
}
)
}
}
<file_sep>import { Component, OnInit } from '@angular/core'
import { PageRoute, RouterExtensions } from '@nativescript/angular'
import { switchMap } from 'rxjs/operators'
import { Car } from '../shared/car.model'
import { CarService } from '../shared/car.service'
@Component({
selector: 'CarDetail',
templateUrl: './car-detail.component.html',
})
export class CarDetailComponent implements OnInit {
private _car: Car
constructor(
private _carService: CarService,
private _pageRoute: PageRoute,
private _routerExtensions: RouterExtensions
) {}
ngOnInit(): void {
this._pageRoute.activatedRoute
.pipe(switchMap((activatedRoute) => activatedRoute.params))
.forEach((params) => {
const carId = params.id
this._car = this._carService.getCarById(carId)
})
}
get car(): Car {
return this._car
}
onBackButtonTap(): void {
this._routerExtensions.backToPreviousPage()
}
onEditButtonTap(): void {
this._routerExtensions.navigate(['/cars/car-detail-edit', this._car.id], {
animated: true,
transition: {
name: 'slideTop',
duration: 200,
curve: 'ease',
},
})
}
}
<file_sep>const sveltePreprocess = require('svelte-preprocess')
const svelteNativePreprocessor = require('svelte-native-preprocessor')
module.exports = {
compilerOptions: {
namespace: "foreign"
},
preprocess: [ sveltePreprocess(), svelteNativePreprocessor() ]
}<file_sep>import { Injectable } from '@angular/core'
export interface DataItem {
id: number
name: string
description: string
}
@Injectable({
providedIn: 'root',
})
export class DataService {
private items = new Array<DataItem>(
{
id: 1,
name: 'Item 1',
description: 'Description for Item 1',
},
{
id: 2,
name: 'Item 2',
description: 'Description for Item 2',
},
{
id: 3,
name: 'Item 3',
description: 'Description for Item 3',
},
{
id: 4,
name: 'Item 4',
description: 'Description for Item 4',
},
{
id: 5,
name: 'Item 5',
description: 'Description for Item 5',
},
{
id: 6,
name: 'Item 6',
description: 'Description for Item 6',
},
{
id: 7,
name: 'Item 7',
description: 'Description for Item 7',
},
{
id: 8,
name: 'Item 8',
description: 'Description for Item 8',
},
{
id: 9,
name: 'Item 9',
description: 'Description for Item 9',
},
{
id: 10,
name: 'Item 10',
description: 'Description for Item 10',
},
{
id: 11,
name: 'Item 11',
description: 'Description for Item 11',
},
{
id: 12,
name: 'Item 12',
description: 'Description for Item 12',
},
{
id: 13,
name: 'Item 13',
description: 'Description for Item 13',
},
{
id: 14,
name: 'Item 14',
description: 'Description for Item 14',
},
{
id: 15,
name: 'Item 15',
description: 'Description for Item 15',
},
{
id: 16,
name: 'Item 16',
description: 'Description for Item 16',
},
{
id: 17,
name: 'Item 17',
description: 'Description for Item 17',
},
{
id: 18,
name: 'Item 18',
description: 'Description for Item 18',
},
{
id: 19,
name: 'Item 19',
description: 'Description for Item 19',
},
{
id: 20,
name: 'Item 20',
description: 'Description for Item 20',
}
)
getItems(): Array<DataItem> {
return this.items
}
getItem(id: number): DataItem {
return this.items.filter((item) => item.id === id)[0]
}
}
<file_sep>import { ListSelectorViewModel } from './list-selector-view-model'
let viewModel
export function onShownModally(args) {
const page = args.object
viewModel = new ListSelectorViewModel(args.context, args.closeCallback)
page.bindingContext = viewModel
}
export function onItemSelected(args) {
viewModel.selectItem(args.index)
}
export function onCancelButtonTap() {
viewModel.cancelSelection()
}
<file_sep>import { NavigatedData, Page } from '@nativescript/core'
import { SearchViewModel } from './search-view-model'
export function onNavigatingTo(args: NavigatedData) {
const page = <Page>args.object
page.bindingContext = new SearchViewModel()
}
<file_sep>
NOTE: This guide assumes that you have a working version of the master / detail NativeScript app set up locally.
### Add Firebase to your app
- Follow the [Firebase documentation](https://firebase.google.com/docs/android/setup#manually_add_firebase) to add Firebase to your app (you will need a Firebase project and a Firebase configuration file for your iOS / Android app).
- When prompted for your app's package name make sure it matches the appid generated for your NativeScript app (open the root `package.json` for your app in your favorite editor and find the "nativescript" node there -- the "id" child node is your appid)
- Download the generated Firebase configuration files and replace the existing `app/App_Resources/iOS/GoogleService-Info.plist` and `app/App_Resources/Android/google-services.json` in your app (for iOS and Android respectively)
- In Firebase go to project settings (the settings button is to the right of the **Overview** side menu item), copy the **Project ID** and replace the one in `app/shared/config.ts` with it. For example if your Project ID is **my-awesome-project-80fbb**, the updated config.ts will look like:
```typescript
export class Config {
static firebaseBucket = "gs://my-awesome-project-80fbb.appspot.com/";
}
```
### Set up your Firebase sample data
- Follow the [Firebase documentation](https://support.google.com/firebase/answer/6386780?hl=en#import) to import the [sample JSON data](https://github.com/NativeScript/nativescript-app-templates/blob/master/packages/template-master-detail-ng/tools/firebase/car-rental-export-public.json) to your Firebase project
- Default database security rules in Firebase require users to be authenticated but [for simplicity] the current version of the NativeScript master / detail app does not provide built-in support for authentication / authorization. Follow the [Firebase documentation](https://firebase.google.com/docs/database/security/quickstart) to replace the default rules (DO NOT use this ruleset for a production app):
```json
{
"rules": {
"cars": {
".read": true,
".write": true
}
}
}
```
- Default storage security rules in Firebase require users to be authenticated in order to upload new content (the master / detail "edit" functionality supports uploading new images to Firebase). Follow the [Firebase documentation](https://firebase.google.com/docs/storage/security/start) to replace the default storage security rules as [for simplicity] the master / detail app does not implement authentication / authorization in the current version (DO NOT use this ruleset for a production app):
```
service firebase.storage {
match /b/{bucket}/o {
match /cars/{allPaths=**} {
allow read,write;
}
}
}
```
- You need to comment out the readonly section in this car detail edit page and uncomment the actual code: https://github.com/NativeScript/nativescript-app-templates/blob/master/packages/template-master-detail-ts/app/cars/car-detail-edit-page/car-detail-edit-page.ts
<file_sep>import { NgModule, NO_ERRORS_SCHEMA } from '@angular/core'
import { NativeScriptCommonModule, NativeScriptFormsModule } from '@nativescript/angular'
import { NativeScriptUIListViewModule } from 'nativescript-ui-listview/angular'
import { CarDetailEditComponent } from './car-detail-edit/car-detail-edit.component'
import { MyImageAddRemoveComponent } from './car-detail-edit/my-image-add-remove/my-image-add-remove.component'
import { MyListSelectorModalViewComponent } from './car-detail-edit/my-list-selector/my-list-selector-modal-view.component'
import { MyListSelectorComponent } from './car-detail-edit/my-list-selector/my-list-selector.component'
import { CarDetailComponent } from './car-detail/car-detail.component'
import { CarListComponent } from './car-list.component'
import { CarsRoutingModule } from './cars-routing.module'
@NgModule({
imports: [
CarsRoutingModule,
NativeScriptCommonModule,
NativeScriptFormsModule,
NativeScriptUIListViewModule,
],
declarations: [
CarListComponent,
CarDetailComponent,
CarDetailEditComponent,
MyListSelectorComponent,
MyListSelectorModalViewComponent,
MyImageAddRemoveComponent,
],
entryComponents: [MyListSelectorModalViewComponent],
providers: [],
schemas: [NO_ERRORS_SCHEMA],
})
export class CarsModule {}
<file_sep># NativeScript with Angular Drawer Navigation Template
App templates help you jump start your native cross-platform apps with built-in UI elements and best practices. Save time writing boilerplate code over and over again when you create new apps.
App template featuring a RadSideDrawer component for navigation. The RadSideDrawer component is part of [Progress NativeScript UI](https://github.com/telerik/nativescript-ui-feedback).
<img src="/packages/template-drawer-navigation-ng/tools/assets/phone-drawer-ios.png" height="400" /> <img src="/packages/template-drawer-navigation-ng/tools/assets/phone-drawer-android.png" height="400" />
## Key Features
- Side drawer navigation
- Five blank pages hooked to the drawer navigation
- Customizable theme
- UX and development best practices
- Easy to understand code
## Quick Start
Execute the following command to create an app from this template:
``` shell
ns create my-drawer-ng --template @nativescript/template-drawer-navigation-ng
```
> Note: This command will create a new NativeScript app that uses the latest version of this template published to [npm](https://www.npmjs.com/package/@nativescript/template-drawer-navigation-ng).
If you want to create a new app that uses the source of the template from the `master` branch, you can execute the following:
``` shell
# clone nativescript-app-templates monorepo locally
git clone [email protected]:NativeScript/nativescript-app-templates.git
# create app template from local source (all templates are in the 'packages' subfolder of the monorepo)
ns create my-drawer-ng --template nativescript-app-templates/packages/template-drawer-navigation-ng
```
**NB:** Please, have in mind that the master branch may refer to dependencies that are not on NPM yet!
## Walkthrough
### Architecture
The RadSideDrawer component is set up as an application root view in:
- `/src/app/app-component` - sets up the side drawer content and defines a page router outlet for the pages.
RadSideDrawer has the following component structure:
- `RadSideDrawer` - The component to display a drawer on the page.
- `tkDrawerContent` directive - Marks the component that will hold the drawer content.
- `tkMainContent` directive - Marks the component that will hold the app main content.
There are five blank components located in these folders:
- `/src/app/browse/browse.component`
- `/src/app/featured/featured.component`
- `/src/app/home/home.component`
- `/src/app/search/search.component`
- `/src/app/settings/settings.component`
### Styling
This template is set up to use SASS for styling. All classes used are based on the {N} core theme – consult the [documentation](https://github.com/NativeScript/theme) to understand how to customize it.
It has 3 global style files that are located at the root of the app folder:
- `/src/_app-common.scss` - the global common style sheet. These style rules are applied to both Android and iOS.
- `/src/app.android.scss` - the global Android style sheet. These style rules are applied to Android only.
- `/src/app.ios.scss` - the global iOS style sheet. These style rules are applied to iOS only.
## Get Help
The NativeScript framework has a vibrant community that can help when you run into problems.
Try [joining the NativeScript community Discord](https://nativescript.org/discord). The Discord channel is a great place to get help troubleshooting problems, as well as connect with other NativeScript developers.
If you have found an issue with this template, please report the problem in the [NativeScript repository](https://github.com/NativeScript/NativeScript/issues).
## Contributing
We love PRs, and accept them gladly. Feel free to propose changes and new ideas. We will review and discuss, so that they can be accepted and better integrated.
<file_sep>import { BrowseViewModel } from './browse-view-model'
export function onNavigatingTo(args) {
const component = args.object
component.bindingContext = new BrowseViewModel()
}
<file_sep>import { fromObject } from '@nativescript/core'
export function HomeViewModel() {
const viewModel = fromObject({
/* Add your view model properties here */
})
return viewModel
}
<file_sep>// @ts-check
const fs = require('fs');
const path = require('path');
const cpy = require('cpy')
const SHARED_PATH = path.resolve(__dirname, './shared')
const PACKAGES_PATH = path.resolve(__dirname, './packages')
const entries = fs.readdirSync(PACKAGES_PATH, {
withFileTypes: true
})
for(const entry of entries) {
if(!entry.isDirectory()) {
continue;
}
prepareTemplate(path.resolve(PACKAGES_PATH, entry.name))
}
function prepareTemplate(templatePath) {
console.log('processing ', templatePath)
// Copy shared resources into template
cpy(`**/*`, templatePath, {
cwd: SHARED_PATH,
overwrite: false,
dot: true,
parents: true
})
}<file_sep>import { Injectable, NgZone } from '@angular/core'
import { Observable, throwError } from 'rxjs'
import { catchError } from 'rxjs/operators'
import ApiService from "~/services/api.service";
import { Car } from './car.model'
const editableProperties = [
'doors',
'imageUrl',
'luggage',
'name',
'price',
'seats',
'transmission',
'class',
]
/* ***********************************************************
* This is the master detail data service. It handles all the data operations
* of retrieving and updating the data. In this case, it is connected to Firebase and
* is using the {N} Firebase plugin. Learn more about it here:
* https://github.com/EddyVerbruggen/nativescript-plugin-firebase
* The {N} Firebase plugin needs some initialization steps before the app starts.
* Check out how it is imported in the main.ts file and the actual script in /shared/firebase.common.ts file.
*************************************************************/
@Injectable({
providedIn: 'root',
})
export class CarService {
private static cloneUpdateModel(car: Car): object {
return editableProperties.reduce((a, e) => ((a[e] = car[e]), a), {})
}
private _cars: Array<Car> = []
constructor(private _ngZone: NgZone) {}
getCarById(id: string): Car {
if (!id) {
return
}
return this._cars.filter((car) => {
return car.id === id
})[0]
}
load(): Observable<any> {
return new Observable((observer: any) => {
const path = 'cars'
const onValueEvent = (snapshot: any) => {
this._ngZone.run(() => {
const results = this.handleSnapshot(snapshot)
observer.next(results)
})
}
ApiService.addValueEventListener(onValueEvent, `/${path}`)
}).pipe(catchError(this.handleErrors))
}
update(carModel: Car): Promise<any> {
const updateModel = CarService.cloneUpdateModel(carModel)
return ApiService.update('/cars/' + carModel.id, updateModel)
}
uploadImage(remoteFullPath: string, localFullPath: string): Promise<any> {
return ApiService.uploadFile({
localFullPath,
remoteFullPath,
onProgress: null,
})
}
private handleSnapshot(data: any): Array<Car> {
this._cars = []
if (data) {
for (const id in data) {
if (data.hasOwnProperty(id)) {
this._cars.push(new Car(data[id]))
}
}
}
return this._cars
}
private handleErrors(error: Response): Observable<never> {
return throwError(error)
}
}
<file_sep>import { RadSideDrawer } from 'nativescript-ui-sidedrawer'
import { Application, EventData, NavigatedData, Page } from '@nativescript/core'
import { SearchViewModel } from './search-view-model'
export function onNavigatingTo(args: NavigatedData) {
const page = <Page>args.object
page.bindingContext = new SearchViewModel()
}
export function onDrawerButtonTap(args: EventData) {
const sideDrawer = <RadSideDrawer>Application.getRootView()
sideDrawer.showDrawer()
}
<file_sep>import { Component } from '@angular/core'
import { ModalDialogParams } from '@nativescript/angular'
@Component({
selector: 'MyListSelectorModalView',
templateUrl: './my-list-selector-modal-view.component.html',
styleUrls: ['./my-list-selector-modal-view.component.scss'],
})
export class MyListSelectorModalViewComponent {
private _items: Array<any>
private _selectedIndex: number
private _title: string
constructor(private _params: ModalDialogParams) {
this._title = _params.context.title
this._selectedIndex = _params.context.selectedIndex
this._items = []
for (let i = 0; i < _params.context.items.length; i++) {
this._items.push({
value: _params.context.items[i],
isSelected: i === this._selectedIndex ? true : false,
})
}
}
onItemSelected(args): void {
const oldSelectedItem = this._items[this._selectedIndex]
oldSelectedItem.isSelected = false
const newSelectedItem = this._items[args.index]
newSelectedItem.isSelected = true
this._selectedIndex = args.index
this._params.closeCallback(newSelectedItem.value)
}
onCancelButtonTap(): void {
this._params.closeCallback(null)
}
get items(): Array<any> {
return this._items
}
get title(): string {
return this._title
}
}
<file_sep># NativeScript App Templates
App templates help you jump start your native cross-platform apps with built-in UI elements and best practices. Save time writing boilerplate code over and over again when you create new apps.
This monorepo contains the following NativeScript app templates:
- Hello World ([JavaScript](/packages/template-hello-world), [TypeScript](/packages/template-hello-world-ts), and [Angular](/packages/template-hello-world-ng))
- Blank ([JavaScript](/packages/template-blank), [TypeScript](/packages/template-blank-ts), [Angular](/packages/template-blank-ng), [Vue](/packages/template-blank-vue), [React](/packages/template-blank-react), and [Svelte](/packages/template-blank-svelte))
- Drawer Navigation ([JavaScript](/packages/template-drawer-navigation), [TypeScript](/packages/template-drawer-navigation-ts), [Angular](/packages/template-drawer-navigation-ng), and [Vue](/packages/template-drawer-navigation-vue))
- Tab Navigation ([JavaScript](/packages/template-tab-navigation), [TypeScript](/packages/template-tab-navigation-ts), [Angular](/packages/template-tab-navigation-ng), and [Vue](/packages/template-tab-navigation-vue))
- Master Detail with Firebase ([JavaScript](/packages/template-master-detail), [TypeScript](/packages/template-master-detail-ts), [Angular](/packages/template-master-detail-ng), and [Vue](/packages/template-master-detail-vue))
- Master Detail with Kinvey ([JavaScript](/packages/template-master-detail-kinvey), [TypeScript](/packages/template-master-detail-kinvey-ts), and [Angular](/packages/template-master-detail-kinvey-ng))
## Get Help
The NativeScript framework has a vibrant community that can help when you run into problems.
Try [joining the NativeScript community Discord](https://nativescript.org/discord). The Discord channel is a great place to get help troubleshooting problems, as well as connect with other NativeScript developers.
If you have found an issue with this template, please report the problem in the [NativeScript repository](https://github.com/NativeScript/NativeScript/issues).
## Contributing
We love PRs, and accept them gladly. Feel free to propose changes and new ideas. We will review and discuss, so that they can be accepted and better integrated.
## Releasing
The templates are automatically released (CI) once they are merged to the main branch as long as the `package.json` version has been updated. Manual releases are not recommended, just bumping the version & pushing to the main branch is enough.
It is recommended to only bump the patch version when making changes - minor/major versions should generally be kep in sync with the NativeScript version (CLI).
<!-- This monorepo uses Lerna to manage packages and their releases.
Install the dependencies in the root of the repo with
```bash
$ npm i # or yarn
```
To list which packages have been changed since the last release
```bash
$ npx lerna changed
```
This will print something like this
```bash
lerna notice cli v3.14.2
lerna info versioning independent
lerna info Looking for changed packages since [email protected]
lerna info ignoring diff in paths matching [ 'ignored-file', '*.md' ]
tns-template-hello-world-ng
lerna success found 1 package ready to publish
```
To manually specify version for each package interactively run
```bash
$ npx lerna version
```
This should prompt the new version number for every changed package.
To release the packages, run
```bash
$ npx lerna publish
```
If you want to bump and publish at the same time,
```bash
$ npx lerna publish
```
will also prompt the new version interactively and then publish the package to npm.
-->
<file_sep>/**
* A record of the navigation params for each route in your app.
*/
export type MainStackParamList = {
One?: {};
Two: {
message: string;
};
};
<file_sep># NativeScript Core Blank Template
App templates help you jump start your native cross-platform apps with built-in UI elements and best practices. Save time writing boilerplate code over and over again when you create new apps.
## Quick Start
Execute the following command to create an app from this template:
```
ns create my-blank-js --template @nativescript/template-blank
```
> Note: This command will create a new NativeScript app that uses the latest version of this template published to [npm](https://www.npmjs.com/package/@nativescript/template-blank).
If you want to create a new app that uses the source of the template from the `master` branch, you can execute the following:
```
# clone nativescript-app-templates monorepo locally
git clone <EMAIL>:NativeScript/nativescript-app-templates.git
# create app template from local source (all templates are in the 'packages' subfolder of the monorepo)
ns create my-blank-js --template nativescript-app-templates/packages/template-blank
```
**NB:** Please, have in mind that the master branch may refer to dependencies that are not on NPM yet!
## Walkthrough
### Architecture
The application root module:
- `/app/app-root` - sets up a Frame that lets you navigate between pages.
There is a single blank page module that sets up an empty layout:
- `/app/home/home-page`
**Home** page has the following components:
- `ActionBar` - It holds the title of the page.
- `GridLayout` - The main page layout that should contains all the page content.
## Get Help
The NativeScript framework has a vibrant community that can help when you run into problems.
Try [joining the NativeScript community Discord](https://nativescript.org/discord). The Discord channel is a great place to get help troubleshooting problems, as well as connect with other NativeScript developers.
If you have found an issue with this template, please report the problem in the [NativeScript repository](https://github.com/NativeScript/NativeScript/issues).
## Contributing
We love PRs, and accept them gladly. Feel free to propose changes and new ideas. We will review and discuss, so that they can be accepted and better integrated.
<file_sep>/*
In NativeScript, a file with the same name as an XML file is known as
a code-behind file. The code-behind is a great place to place your view
logic, and to set up your page’s data binding.
*/
import { NavigatedData, Page } from '@nativescript/core'
import { HomeViewModel } from './home-view-model'
export function onNavigatingTo(args: NavigatedData) {
const page = <Page>args.object
page.bindingContext = new HomeViewModel()
}
<file_sep>import * as React from 'react';
import * as ReactNativeScript from 'react-nativescript';
import { MainStack } from './components/MainStack';
// In NativeScript, the app.ts file is the entry point to your application. You
// can use this file to perform app-level initialization, but the primary
// purpose of the file is to pass control to the app’s first module.
// Controls react-nativescript log verbosity.
// - true: all logs;
// - false: only error logs.
Object.defineProperty(global, '__DEV__', { value: false });
ReactNativeScript.start(React.createElement(MainStack, {}, null));
// Do not place any code after the application has been started as it will not
// be executed on iOS.
<file_sep>import { Observable, Subscription, throwError } from 'rxjs'
import { catchError } from 'rxjs/operators'
import { Car } from './car-model'
import ApiService from "~/services/api.service";
const editableProperties = [
'doors',
'imageUrl',
'luggage',
'name',
'price',
'seats',
'transmission',
'class',
]
export class CarService {
static getInstance(): CarService {
return CarService._instance
}
private static _instance: CarService = new CarService()
private static cloneUpdateModel(car: Car): object {
return editableProperties.reduce((a, e) => ((a[e] = car[e]), a), {})
}
private _subscriptionMap = new Map<string, Subscription>()
private _cars: Array<Car> = []
constructor() {
if (CarService._instance) {
throw new Error('Use CarService.getInstance() instead of new.')
}
CarService._instance = this
}
getSubscription(key: string): Subscription {
return this._subscriptionMap.get(key)
}
setSubscription(key: string, value: Subscription): void {
this._subscriptionMap.set(key, value)
}
load(): Observable<any> {
return new Observable((observer: any) => {
const path = 'cars'
const onValueEvent = (snapshot: any) => {
const results = this.handleSnapshot(snapshot)
observer.next(results)
}
ApiService.addValueEventListener(onValueEvent, `/${path}`)
}).pipe(catchError(this.handleErrors))
}
update(carModel: Car): Promise<any> {
const updateModel = CarService.cloneUpdateModel(carModel)
return ApiService.update(`/cars/${carModel.id}`, updateModel)
}
uploadImage(remoteFullPath: string, localFullPath: string): Promise<any> {
return ApiService.uploadFile({
localFullPath,
remoteFullPath,
onProgress: null,
})
}
private handleSnapshot(data: any): Array<Car> {
this._cars = []
if (data) {
for (const id in data) {
if (data.hasOwnProperty(id)) {
this._cars.push(new Car(data[id]))
}
}
}
return this._cars
}
private handleErrors(error: Response): Observable<any> {
return throwError(error)
}
}
<file_sep>import { RadSideDrawer } from 'nativescript-ui-sidedrawer'
import { Application, EventData, Frame, GridLayout } from '@nativescript/core'
import { AppRootViewModel } from './app-root-view-model'
export function onLoaded(args: EventData): void {
const drawerComponent = <RadSideDrawer>args.object
drawerComponent.bindingContext = new AppRootViewModel()
}
export function onNavigationItemTap(args: EventData): void {
const component = <GridLayout>args.object
const componentRoute = component.get('route')
const componentTitle = component.get('title')
const bindingContext = <AppRootViewModel>component.bindingContext
bindingContext.selectedPage = componentTitle
Frame.topmost().navigate({
moduleName: componentRoute,
transition: {
name: 'fade',
},
})
const drawerComponent = <RadSideDrawer>Application.getRootView()
drawerComponent.closeDrawer()
}
<file_sep>import { Observable } from '@nativescript/core'
function getMessage(counter) {
if (counter <= 0) {
return 'Hoorraaay! You unlocked the NativeScript clicker achievement!'
} else {
return `${counter} taps left`
}
}
export function createViewModel() {
const viewModel = new Observable()
viewModel.counter = 42
viewModel.message = getMessage(viewModel.counter)
viewModel.onTap = () => {
viewModel.counter--
viewModel.set('message', getMessage(viewModel.counter))
}
return viewModel
}
<file_sep>import { EventData, Dialogs, Frame, GridLayout, NavigatedData, Page } from '@nativescript/core'
import { CarDetailEditViewModel } from './car-detail-edit-view-model'
export function onNavigatingTo(args: NavigatedData): void {
if (args.isBackNavigation) {
return
}
const page = <Page>args.object
page.bindingContext = new CarDetailEditViewModel(page.navigationContext)
}
export function onCancelButtonTap(args: EventData): void {
Frame.topmost().goBack()
}
export function onDoneButtonTap(args: EventData): void {
/* ***********************************************************
* By design this app is set up to work with read-only sample data.
* Follow the steps in the "Firebase database setup" section in app/readme.md file
* and uncomment the code block below to make it editable.
*************************************************************/
/* ***********************************************************
const actionItem = <ActionItem>args.object;
const bindingContext = <CarDetailEditViewModel>actionItem.bindingContext;
bindingContext.saveChanges()
.then(() => Frame.topmost().navigate({
moduleName: "cars/cars-list-page",
clearHistory: true,
animated: true,
transition: {
name: "slideBottom",
duration: 200,
curve: "ease"
}
}))
.catch((errorMessage: any) =>
Dialogs.alert({ title: "Oops!", message: "Something went wrong. Please try again.", okButtonText: "Ok" }));
*************************************************************/
/* ***********************************************************
* Comment out the code block below if you made the app editable.
*************************************************************/
const readOnlyMessage =
'Check out the "Firebase database setup" section in the readme file to make it editable.'
const queue = Promise.resolve()
queue
.then(() =>
Dialogs.alert({
title: 'Read-Only Template!',
message: readOnlyMessage,
okButtonText: 'Ok',
})
)
.then(() =>
Frame.topmost().navigate({
moduleName: 'cars/cars-list-page',
clearHistory: true,
animated: true,
transition: {
name: 'slideBottom',
duration: 200,
curve: 'ease',
},
})
)
}
export function onSelectorTap(args: EventData): void {
const gridLayout = <GridLayout>args.object
const tag = gridLayout.get('tag')
const bindingContext = <CarDetailEditViewModel>gridLayout.bindingContext
const selectedValue = bindingContext.car[tag]
const context = { tag, selectedValue }
const modalPagePath = 'cars/list-selector-modal-page/list-selector-modal-page'
const page = <Page>gridLayout.page
page.showModal(modalPagePath, {
context,
closeCallback: (value: string) => {
if (value) {
bindingContext.car[tag] = value
}
},
fullscreen: false,
})
}
export function onImageAddRemoveTap(args: EventData): void {
const gridLayout = <GridLayout>args.object
const bindingContext = <CarDetailEditViewModel>gridLayout.bindingContext
bindingContext.onImageAddRemove()
}
<file_sep>import { Observable } from '@nativescript/core'
import { SelectedPageService } from '../shared/selected-page-service'
export class BrowseViewModel extends Observable {
constructor() {
super()
SelectedPageService.getInstance().updateSelectedPage('Browse')
}
}
<file_sep>export class Car {
id: string
name: string
hasAC: boolean
seats: string
luggage: number
class: string
doors: number
price: number
transmission: string
imageUrl: string
imageStoragePath: string
constructor(options: any) {
this.id = options.id
this.name = options.name
this.hasAC = options.hasAC
this.seats = options.seats
this.luggage = Number(options.luggage)
this.class = options.class
this.doors = Number(options.doors)
this.price = Number(options.price)
this.transmission = options.transmission
this.imageUrl = options.imageUrl
this.imageStoragePath = options.imageStoragePath
}
}
<file_sep>import { fromObject } from '@nativescript/core'
export function HomeItemsViewModel() {
const viewModel = fromObject({
items: [
{
name: 'Item 1',
description: 'Description for Item 1',
},
{
name: 'Item 2',
description: 'Description for Item 2',
},
{
name: 'Item 3',
description: 'Description for Item 3',
},
{
name: 'Item 4',
description: 'Description for Item 4',
},
{
name: 'Item 5',
description: 'Description for Item 5',
},
{
name: 'Item 6',
description: 'Description for Item 6',
},
{
name: 'Item 7',
description: 'Description for Item 7',
},
{
name: 'Item 8',
description: 'Description for Item 8',
},
{
name: 'Item 9',
description: 'Description for Item 9',
},
{
name: 'Item 10',
description: 'Description for Item 10',
},
{
name: 'Item 11',
description: 'Description for Item 11',
},
{
name: 'Item 12',
description: 'Description for Item 12',
},
{
name: 'Item 13',
description: 'Description for Item 13',
},
{
name: 'Item 14',
description: 'Description for Item 14',
},
{
name: 'Item 15',
description: 'Description for Item 15',
},
{
name: 'Item 16',
description: 'Description for Item 16',
},
],
})
return viewModel
}
<file_sep>/*
In NativeScript, the app.js file is the entry point to your application.
You can use this file to perform app-level initialization, but the primary
purpose of the file is to pass control to the app’s first module.
*/
import { Application } from '@nativescript/core';
Application.run({ moduleName: 'app-root' })
/*
Do not place any code after the application has been started as it will not
be executed on iOS.
*/
<file_sep>import { Component, OnInit } from '@angular/core'
import { PageRoute, RouterExtensions } from '@nativescript/angular'
import { switchMap } from 'rxjs/operators'
import { Dialogs } from '@nativescript/core'
import { CarEditService } from '../shared/car-edit.service'
import { Car } from '../shared/car.model'
import { CarService } from '../shared/car.service'
import { carClassList, carDoorList, carSeatList, carTransmissionList } from './constants'
@Component({
selector: 'CarDetailEdit',
templateUrl: './car-detail-edit.component.html',
styleUrls: ['./car-detail-edit.component.scss'],
})
export class CarDetailEditComponent implements OnInit {
private _car: Car
private _carClassOptions: Array<string> = []
private _carDoorOptions: Array<number> = []
private _carSeatOptions: Array<string> = []
private _carTransmissionOptions: Array<string> = []
private _isCarImageDirty: boolean = false
private _isUpdating: boolean = false
constructor(
private _carService: CarService,
private _carEditService: CarEditService,
private _pageRoute: PageRoute,
private _routerExtensions: RouterExtensions
) {}
ngOnInit(): void {
this.initializeEditOptions()
this._pageRoute.activatedRoute
.pipe(switchMap((activatedRoute) => activatedRoute.params))
.forEach((params) => {
const carId = params.id
this._car = this._carEditService.startEdit(carId)
})
}
get isUpdating(): boolean {
return this._isUpdating
}
get car(): Car {
return this._car
}
get pricePerDay(): number {
return this._car.price
}
set pricePerDay(value: number) {
// force iOS UISlider to work with discrete steps
this._car.price = Math.round(value)
}
get luggageValue(): number {
return this._car.luggage
}
set luggageValue(value: number) {
// force iOS UISlider to work with discrete steps
this._car.luggage = Math.round(value)
}
get carClassOptions(): Array<string> {
return this._carClassOptions
}
get carDoorOptions(): Array<number> {
return this._carDoorOptions
}
get carSeatOptions(): Array<string> {
return this._carSeatOptions
}
get carTransmissionOptions(): Array<string> {
return this._carTransmissionOptions
}
get carImageUrl(): string {
return this._car.imageUrl
}
set carImageUrl(value: string) {
this._car.imageUrl = value
this._isCarImageDirty = true
}
onCancelButtonTap(): void {
this._routerExtensions.backToPreviousPage()
}
onDoneButtonTap(): void {
/* ***********************************************************
* By design this app is set up to work with read-only sample data.
* Follow the steps in the "Firebase database setup" section in app/readme.md file
* and uncomment the code block below to make it editable.
*************************************************************/
/* ***********************************************************
let queue = Promise.resolve();
this._isUpdating = true;
if (this._isCarImageDirty && this._car.imageUrl) {
queue = queue
.then(() => this._carService.uploadImage(this._car.imageStoragePath, this._car.imageUrl))
.then((uploadedFile: any) => {
this._car.imageUrl = uploadedFile.url;
});
}
queue.then(() => this._carService.update(this._car))
.then(() => {
this._isUpdating = false;
this._routerExtensions.navigate(["/cars"], {
clearHistory: true,
animated: true,
transition: {
name: "slideBottom",
duration: 200,
curve: "ease"
}
});
})
.catch((errorMessage: any) => {
this._isUpdating = false;
Dialogs.alert({ title: "Oops!", message: "Something went wrong. Please try again.", okButtonText: "Ok" });
});
*************************************************************/
/* ***********************************************************
* Comment out the code block below if you made the app editable.
*************************************************************/
const readOnlyMessage =
'Check out the "Firebase database setup" section in the readme file to make it editable.'
const queue = Promise.resolve()
queue
.then(() =>
Dialogs.alert({
title: 'Read-Only Template!',
message: readOnlyMessage,
okButtonText: 'Ok',
})
)
.then(() =>
this._routerExtensions.navigate(['/cars'], {
clearHistory: true,
animated: true,
transition: {
name: 'slideBottom',
duration: 200,
curve: 'ease',
},
})
)
}
private initializeEditOptions(): void {
for (const classItem of carClassList) {
this._carClassOptions.push(classItem)
}
for (const doorItem of carDoorList) {
this._carDoorOptions.push(doorItem)
}
for (const seatItem of carSeatList) {
this._carSeatOptions.push(seatItem)
}
for (const transmissionItem of carTransmissionList) {
this._carTransmissionOptions.push(transmissionItem)
}
}
}
<file_sep>import { BehaviorSubject, Observable } from 'rxjs'
export class SelectedPageService {
static getInstance(): SelectedPageService {
return SelectedPageService._instance
}
private static _instance: SelectedPageService = new SelectedPageService()
selectedPage$: Observable<string>
private _selectedPageSource: BehaviorSubject<string>
constructor() {
if (SelectedPageService._instance) {
throw new Error('Use SelectedPageService.getInstance() instead of new.')
}
SelectedPageService._instance = this
// Observable selectedPage source
this._selectedPageSource = new BehaviorSubject<string>('Home')
// Observable selectedPage stream
this.selectedPage$ = this._selectedPageSource.asObservable()
}
updateSelectedPage(selectedPage: string) {
this._selectedPageSource.next(selectedPage)
}
}
<file_sep>import { Observable } from '@nativescript/core'
export function ObservableProperty() {
return (target: Observable, propertyKey: string) => {
Object.defineProperty(target, propertyKey, {
get() {
return this['_' + propertyKey]
},
set(value) {
if (this['_' + propertyKey] === value) {
return
}
this['_' + propertyKey] = value
this.notifyPropertyChange(propertyKey, value)
},
enumerable: true,
configurable: true,
})
}
}
<file_sep>/*
In NativeScript, the app.ts file is the entry point to your application.
You can use this file to perform app-level initialization, but the primary
purpose of the file is to pass control to the app’s first page.
*/
import { svelteNativeNoFrame } from 'svelte-native'
import App from './App.svelte'
svelteNativeNoFrame(App, {})
<file_sep># NativeScript TypeScript Template
This template creates a NativeScript app with the NativeScript hello world example,
however, in this template the example is built with TypeScript.
You can create a new app that uses this template with either the `--template` option.
```
ns create my-hello-world-ts --template @nativescript/template-hello-world-ts
```
Or the `--tsc` shorthand.
```
ns create my-hello-world-ts --tsc
```
> Note: Both commands will create a new NativeScript app that uses the latest version of this template published to [npm](https://www.npmjs.com/package/@nativescript/template-hello-world-ts).
If you want to create a new app that uses the source of the template from the `master` branch, you can execute the following:
```
# clone nativescript-app-templates monorepo locally
git clone <EMAIL>:NativeScript/nativescript-app-templates.git
# create app template from local source (all templates are in the 'packages' subfolder of the monorepo)
ns create my-hello-world-ts --template nativescript-app-templates/packages/template-hello-world-ts
```
## Get Help
The NativeScript framework has a vibrant community that can help when you run into problems.
Try [joining the NativeScript community Discord](https://nativescript.org/discord). The Discord channel is a great place to get help troubleshooting problems, as well as connect with other NativeScript developers.
If you have found an issue with this template, please report the problem in the [NativeScript repository](https://github.com/NativeScript/NativeScript/issues).
## Contributing
We love PRs, and accept them gladly. Feel free to propose changes and new ideas. We will review and discuss, so that they can be accepted and better integrated.
<file_sep>// @ts-ignore
import db from "./car-rental-export-public.json"
class ApiService {
private db: any;
constructor() {
this.db = db;
}
async update(path, updateModel) {
const carId = path.split("/").slice(-1)[0];
this.db.cars[carId] = {...this.db.cars[carId], ...updateModel }
}
async uploadFile(params) {
return {url: params.localFullPath}
}
addValueEventListener(onValueEvent, path) {
onValueEvent(this.db.cars)
return {
path: path,
listeners: this.db.cars
}
}
}
export default new ApiService;
<file_sep>import { fromObject } from '@nativescript/core'
export function CarDetailViewModel(carModel) {
const viewModel = fromObject({
car: carModel,
})
return viewModel
}
<file_sep>export class RoundingValueConverter {
toView(value: number): number {
return value
}
toModel(value: number): number {
return Math.round(value)
}
}
<file_sep>import { Injectable } from '@angular/core'
import { Car } from './car.model'
import { CarService } from './car.service'
@Injectable({
providedIn: 'root',
})
export class CarEditService {
private _editModel: Car
constructor(private _carService: CarService) {}
startEdit(id: string): Car {
this._editModel = null
return this.getEditableCarById(id)
}
getEditableCarById(id: string): Car {
if (!this._editModel || this._editModel.id !== id) {
const car = this._carService.getCarById(id)
// get fresh editable copy of car model
this._editModel = new Car(car)
}
return this._editModel
}
}
<file_sep>import { Observable } from '@nativescript/core'
import { ObservableProperty } from '../shared/observable-property-decorator'
import { SelectedPageService } from '../shared/selected-page-service'
export class AppRootViewModel extends Observable {
@ObservableProperty() selectedPage: string
constructor() {
super()
SelectedPageService.getInstance().selectedPage$.subscribe(
(selectedPage: string) => (this.selectedPage = selectedPage)
)
}
}
<file_sep>import { Component, forwardRef, Input, Output } from '@angular/core'
import { ControlValueAccessor, NG_VALUE_ACCESSOR } from '@angular/forms'
import * as imagePicker from '@nativescript/imagepicker'
import { Folder, knownFolders, path, ImageAsset, ImageSource } from '@nativescript/core'
const tempImageFolderName = 'nsimagepicker'
const noop = () => {}
const MY_IMAGE_ADD_REMOVE_CONTROL_VALUE_ACCESSOR = {
provide: NG_VALUE_ACCESSOR,
useExisting: forwardRef(() => MyImageAddRemoveComponent),
multi: true,
}
/* ***********************************************************
* The MyImageAddRemove custom component uses an imagepicker plugin to let the user select
* an image and provides custom logic and design to the process.
*************************************************************/
@Component({
selector: 'MyImageAddRemove',
templateUrl: './my-image-add-remove.component.html',
styleUrls: ['./my-image-add-remove.component.scss'],
providers: [MY_IMAGE_ADD_REMOVE_CONTROL_VALUE_ACCESSOR],
})
export class MyImageAddRemoveComponent implements ControlValueAccessor {
static get imageTempFolder(): Folder {
return knownFolders.temp().getFolder(tempImageFolderName)
}
private static clearImageTempFolder(): void {
MyImageAddRemoveComponent.imageTempFolder.clear()
}
// placeholder for the callback later provided by the ControlValueAccessor
private propagateChange: (_: any) => void = noop
private innerImageUrl: string = ''
get imageUrl(): string {
return this.innerImageUrl
}
set imageUrl(value: string) {
if (this.innerImageUrl !== value) {
this.innerImageUrl = value
this.propagateChange(value)
}
}
// ControlValueAccessor implementation
writeValue(value: string) {
if (this.innerImageUrl !== value) {
this.innerImageUrl = value
}
}
// ControlValueAccessor implementation
registerOnChange(fn: any): void {
this.propagateChange = fn
}
// ControlValueAccessor implementation
registerOnTouched(fn: any): void {}
onImageAddRemoveTap(): void {
if (this.imageUrl) {
this.handleImageChange(null)
return
}
MyImageAddRemoveComponent.clearImageTempFolder()
this.pickImage()
}
pickImage(): void {
const context = imagePicker.create({
mode: 'single',
})
context
.authorize()
.then(() => context.present())
.then((selection) =>
selection.forEach((selectedAsset: ImageAsset) => {
selectedAsset.options.height = 768
ImageSource.fromAsset(selectedAsset).then((imageSource: ImageSource) =>
this.handleImageChange(imageSource)
)
})
)
.catch((errorMessage: any) => console.log(errorMessage))
}
private handleImageChange(source: ImageSource): void {
let raisePropertyChange = true
let tempImagePath = null
if (source) {
tempImagePath = path.join(MyImageAddRemoveComponent.imageTempFolder.path, `${Date.now()}.jpg`)
raisePropertyChange = source.saveToFile(tempImagePath, 'jpeg')
}
if (raisePropertyChange) {
this.imageUrl = tempImagePath
}
}
}
<file_sep>import { View, ItemEventData, NavigatedData, Page } from '@nativescript/core'
import { HomeViewModel } from './home-view-model'
import { Item } from './shared/item'
export function onNavigatingTo(args: NavigatedData) {
const page = <Page>args.object
page.bindingContext = new HomeViewModel()
}
export function onItemTap(args: ItemEventData) {
const view = <View>args.view
const page = <Page>view.page
const tappedItem = <Item>view.bindingContext
page.frame.navigate({
moduleName: 'home/home-item-detail/home-item-detail-page',
context: tappedItem,
animated: true,
transition: {
name: 'slide',
duration: 200,
curve: 'ease',
},
})
}
<file_sep>import { SearchViewModel } from './search-view-model'
export function onNavigatingTo(args) {
const component = args.object
component.bindingContext = new SearchViewModel()
}
<file_sep>import { fromObject } from '@nativescript/core'
import { SelectedPageService } from '../shared/selected-page-service'
export function AppRootViewModel() {
const viewModel = fromObject({
selectedPage: '',
})
SelectedPageService.getInstance().selectedPage$.subscribe((selectedPage) => {
viewModel.selectedPage = selectedPage
})
return viewModel
}
<file_sep>export class VisibilityValueConverter {
toView(value: string): string {
if (value) {
return 'collapsed'
} else {
return 'visible'
}
}
toModel(value: string): string {
return value
}
}
<file_sep>import { fromObject, ObservableArray } from '@nativescript/core'
import { finalize } from 'rxjs/operators'
import { CarService } from './shared/car-service'
const subscriptionKey = 'car-list-view-model'
export function CarsListViewModel() {
const viewModel = fromObject({
cars: new ObservableArray([]),
isLoading: false,
_carService: CarService.getInstance(),
_dataSubscription: null,
load: function () {
if (!this._dataSubscription) {
this.set('isLoading', true)
// we need to be able to unsubscribe from data changes when old page got disposed / inaccessible but
// we do not have equivalent of Angular ngOnInit / ngOnDestroy so it cannot be implicit solution i.e.
// we should explicitly state when to unsubscribe e.g. in the following scenario master list #1 ->
// car detail -> edit car detail -> master list #2 (forward nav with clearHistory)
// we need to unsubscribe the master list #1 view model (as it can never be accessed again)
// if cached subscription exists here, we know it is from a different (previous) instance of the same
// view model and we need to unsubscribe from it
const cachedSubscription = this._carService.getSubscription(subscriptionKey)
if (cachedSubscription) {
cachedSubscription.unsubscribe()
this._carService.setSubscription(subscriptionKey, null)
}
this._dataSubscription = this._carService
.load()
.pipe(finalize(() => this.set('isLoading', false)))
.subscribe((cars) => {
this.set('cars', new ObservableArray(cars))
this.set('isLoading', false)
})
this._carService.setSubscription(subscriptionKey, this._dataSubscription)
}
},
unload: function () {
if (this._dataSubscription) {
this._dataSubscription.unsubscribe()
this._dataSubscription = null
}
},
})
return viewModel
}
| 713261adcc143f409dcb0a1d63ad8b8b4fb2dc3b | [
"Markdown",
"TypeScript",
"JavaScript"
] | 80 | Markdown | NativeScript/nativescript-app-templates | a5e8e3b8d7272f729d8d4c648815f4038054c703 | cb0dce16eff0dc2e2909b24a7d11af646f77183d | |
refs/heads/master | <repo_name>geekmi/NSwag<file_sep>/src/NSwagStudio/ViewModels/ClientGenerators/TypeScriptCodeGeneratorViewModel.cs
//-----------------------------------------------------------------------
// <copyright file="TypeScriptCodeGeneratorViewModel.cs" company="NSwag">
// Copyright (c) <NAME>. All rights reserved.
// </copyright>
// <license>https://github.com/NSwag/NSwag/blob/master/LICENSE.md</license>
// <author><NAME>, <EMAIL></author>
//-----------------------------------------------------------------------
using System;
using System.Linq;
using System.Threading.Tasks;
using System.Windows;
using MyToolkit.Storage;
using NSwag;
using NSwag.CodeGeneration.ClientGenerators;
using NSwag.CodeGeneration.ClientGenerators.TypeScript;
using NSwag.Commands;
namespace NSwagStudio.ViewModels.ClientGenerators
{
public class TypeScriptCodeGeneratorViewModel : ViewModelBase
{
private string _clientCode;
private SwaggerToTypeScriptCommand _command = new SwaggerToTypeScriptCommand();
public bool ShowSettings
{
get { return ApplicationSettings.GetSetting("TypeScriptCodeGeneratorViewModel.ShowSettings", true); }
set { ApplicationSettings.SetSetting("TypeScriptCodeGeneratorViewModel.ShowSettings", value); }
}
/// <summary>Gets the settings.</summary>
public SwaggerToTypeScriptCommand Command
{
get { return _command; }
set
{
if (Set(ref _command, value))
RaiseAllPropertiesChanged();
}
}
/// <summary>Gets the output templates. </summary>
public TypeScriptTemplate[] Templates
{
get { return Enum.GetNames(typeof(TypeScriptTemplate)).Select(t => (TypeScriptTemplate)Enum.Parse(typeof(TypeScriptTemplate), t)).ToArray(); }
}
/// <summary>Gets the async types. </summary>
public OperationGenerationMode[] OperationGenerationModes
{
get { return Enum.GetNames(typeof(OperationGenerationMode)).Select(t => (OperationGenerationMode)Enum.Parse(typeof(OperationGenerationMode), t)).ToArray(); }
}
/// <summary>Gets or sets the client code. </summary>
public string ClientCode
{
get { return _clientCode; }
set { Set(ref _clientCode, value); }
}
public Task GenerateClientAsync(string swaggerData)
{
return RunTaskAsync(async () =>
{
var code = string.Empty;
await Task.Run(async () =>
{
if (!string.IsNullOrEmpty(swaggerData))
{
Command.Input = swaggerData;
code = await Command.RunAsync();
Command.Input = null;
}
});
ClientCode = code;
});
}
public override void HandleException(Exception exception)
{
MessageBox.Show(exception.Message);
}
}
}
<file_sep>/src/NSwagStudio/Views/ClientGenerators/TypeScriptCodeGeneratorView.xaml.cs
using System.Threading.Tasks;
using System.Windows;
using MyToolkit.Mvvm;
using NSwag.CodeGeneration.ClientGenerators.TypeScript;
using NSwag.Commands;
using NSwagStudio.ViewModels;
using NSwagStudio.ViewModels.ClientGenerators;
namespace NSwagStudio.Views.ClientGenerators
{
public partial class TypeScriptCodeGeneratorView : IClientGenerator
{
public TypeScriptCodeGeneratorView(SwaggerToTypeScriptCommand command)
{
InitializeComponent();
ViewModelHelper.RegisterViewModel(Model, this);
Model.Command = command;
}
private TypeScriptCodeGeneratorViewModel Model { get { return (TypeScriptCodeGeneratorViewModel)Resources["ViewModel"]; } }
public string Title { get { return "TypeScript Client"; } }
public Task GenerateClientAsync(string swaggerData)
{
return Model.GenerateClientAsync(swaggerData);
}
public override string ToString()
{
return Title;
}
}
}
<file_sep>/src/NSwag.CodeGeneration/ClientGenerators/CSharp/SwaggerToCSharpGeneratorSettings.cs
//-----------------------------------------------------------------------
// <copyright file="SwaggerToCSharpGeneratorSettings.cs" company="NSwag">
// Copyright (c) <NAME>. All rights reserved.
// </copyright>
// <license>https://github.com/NSwag/NSwag/blob/master/LICENSE.md</license>
// <author><NAME>, <EMAIL></author>
//-----------------------------------------------------------------------
using NJsonSchema.CodeGeneration.CSharp;
namespace NSwag.CodeGeneration.ClientGenerators.CSharp
{
/// <summary>Settings for the <see cref="SwaggerToCSharpGenerator"/>.</summary>
public class SwaggerToCSharpGeneratorSettings : ClientGeneratorBaseSettings
{
/// <summary>Initializes a new instance of the <see cref="SwaggerToCSharpGeneratorSettings"/> class.</summary>
public SwaggerToCSharpGeneratorSettings()
{
ClassName = "{controller}Client";
AdditionalNamespaceUsages = null;
CSharpGeneratorSettings = new CSharpGeneratorSettings();
}
/// <summary>Gets or sets the CSharp generator settings.</summary>
public CSharpGeneratorSettings CSharpGeneratorSettings { get; set; }
/// <summary>Gets or sets the class name of the service client.</summary>
public string ClassName { get; set; }
/// <summary>Gets or sets the full name of the base class.</summary>
public string ClientBaseClass { get; set; }
/// <summary>Gets or sets a value indicating whether to call CreateHttpClientAsync on the base class to create a new HttpClient.</summary>
public bool UseHttpClientCreationMethod { get; set; }
/// <summary>Gets or sets the additional namespace usages.</summary>
public string[] AdditionalNamespaceUsages { get; set; }
}
}<file_sep>/src/NSwag/Commands/SwaggerToCSharpCommand.cs
using System.ComponentModel;
using System.Threading.Tasks;
using NConsole;
using Newtonsoft.Json;
using NJsonSchema.CodeGeneration.CSharp;
using NSwag.CodeGeneration.ClientGenerators;
using NSwag.CodeGeneration.ClientGenerators.CSharp;
using NSwag.Commands.Base;
namespace NSwag.Commands
{
[Description("Generates CSharp client code from a Swagger specification.")]
public class SwaggerToCSharpCommand : InputOutputCommandBase
{
public SwaggerToCSharpCommand()
{
Settings = new SwaggerToCSharpGeneratorSettings();
Namespace = "MyNamespace";
}
[JsonIgnore]
public SwaggerToCSharpGeneratorSettings Settings { get; set; }
[Description("The class name of the generated client.")]
[Argument(Name = "ClassName")]
public string ClassName
{
get { return Settings.ClassName; }
set { Settings.ClassName = value; }
}
[Description("The namespace of the generated classes.")]
[Argument(Name = "Namespace")]
public string Namespace
{
get { return Settings.CSharpGeneratorSettings.Namespace; }
set { Settings.CSharpGeneratorSettings.Namespace = value; }
}
[Description("Specifies whether generate client classes.")]
[Argument(Name = "GenerateClientClasses", DefaultValue = true)]
public bool GenerateClientClasses
{
get { return Settings.GenerateClientClasses; }
set { Settings.GenerateClientClasses = value; }
}
[Description("Specifies whether generate interfaces for the client classes.")]
[Argument(Name = "GenerateClientInterfaces", DefaultValue = false)]
public bool GenerateClientInterfaces
{
get { return Settings.GenerateClientInterfaces; }
set { Settings.GenerateClientInterfaces = value; }
}
[Description("Specifies whether to generate DTO classes.")]
[Argument(Name = "GenerateDtoTypes", DefaultValue = true)]
public bool GenerateDtoTypes
{
get { return Settings.GenerateDtoTypes; }
set { Settings.GenerateDtoTypes = value; }
}
[Description("The client base class (empty for no base class).")]
[Argument(Name = "ClientBaseClass", DefaultValue = "")]
public string ClientBaseClass
{
get { return Settings.ClientBaseClass; }
set { Settings.ClientBaseClass = value; }
}
[Description("Specifies whether to call CreateHttpClientAsync on the base class to create a new HttpClient.")]
[Argument(Name = "UseHttpClientCreationMethod", DefaultValue = false)]
public bool UseHttpClientCreationMethod
{
get { return Settings.UseHttpClientCreationMethod; }
set { Settings.UseHttpClientCreationMethod = value; }
}
[Description("The operation generation mode ('SingleClientFromOperationId' or 'MultipleClientsFromPathSegments').")]
[Argument(Name = "OperationGenerationMode", DefaultValue = OperationGenerationMode.SingleClientFromOperationId)]
public OperationGenerationMode OperationGenerationMode
{
get { return Settings.OperationGenerationMode; }
set { Settings.OperationGenerationMode = value; }
}
[Description("Specifies whether a required property must be defined in JSON (sets Required.Always when the property is required).")]
[Argument(Name = "RequiredPropertiesMustBeDefined", DefaultValue = true)]
public bool RequiredPropertiesMustBeDefined
{
get { return Settings.CSharpGeneratorSettings.RequiredPropertiesMustBeDefined; }
set { Settings.CSharpGeneratorSettings.RequiredPropertiesMustBeDefined = value; }
}
[Description("The additional namespace usages.")]
[Argument(Name = "AdditionalNamespaceUsages", DefaultValue = null)]
public string[] AdditionalNamespaceUsages
{
get { return Settings.AdditionalNamespaceUsages; }
set { Settings.AdditionalNamespaceUsages = value; }
}
[Description("The date time .NET type (default: 'DateTime').")]
[Argument(Name = "DateTimeType", DefaultValue = "DateTime")]
public string DateTimeType
{
get { return Settings.CSharpGeneratorSettings.DateTimeType; }
set { Settings.CSharpGeneratorSettings.DateTimeType = value; }
}
[Description("The generic array .NET type (default: 'ObservableCollection').")]
[Argument(Name = "ArrayType", DefaultValue = "ObservableCollection")]
public string ArrayType
{
get { return Settings.CSharpGeneratorSettings.ArrayType; }
set { Settings.CSharpGeneratorSettings.ArrayType = value; }
}
[Description("The generic dictionary .NET type (default: 'Dictionary').")]
[Argument(Name = "DictionaryType", DefaultValue = "Dictionary")]
public string DictionaryType
{
get { return Settings.CSharpGeneratorSettings.DictionaryType; }
set { Settings.CSharpGeneratorSettings.DictionaryType = value; }
}
public override async Task RunAsync(CommandLineProcessor processor, IConsoleHost host)
{
var output = await RunAsync();
WriteOutput(host, output);
}
public async Task<string> RunAsync()
{
var clientGenerator = new SwaggerToCSharpGenerator(InputSwaggerService, Settings);
return clientGenerator.GenerateFile();
}
}
}<file_sep>/src/NSwagStudio/Views/ClientGenerators/SwaggerGeneratorView.xaml.cs
using System;
using System.Threading.Tasks;
using System.Windows;
using NSwag;
using NSwagStudio.ViewModels.ClientGenerators;
namespace NSwagStudio.Views.ClientGenerators
{
public partial class SwaggerGeneratorView : IClientGenerator
{
public SwaggerGeneratorView()
{
InitializeComponent();
}
public string Title { get { return "Swagger Specification"; } }
private SwaggerGeneratorViewModel Model { get { return (SwaggerGeneratorViewModel)Resources["ViewModel"]; } }
public Task GenerateClientAsync(string swaggerData)
{
return Model.GenerateClientAsync(swaggerData);
}
}
}
<file_sep>/src/NSwag.CodeGeneration/ClientGenerators/TypeScript/SwaggerToTypeScriptGeneratorSettings.cs
//-----------------------------------------------------------------------
// <copyright file="SwaggerToTypeScriptGeneratorSettings.cs" company="NSwag">
// Copyright (c) <NAME>. All rights reserved.
// </copyright>
// <license>https://github.com/NSwag/NSwag/blob/master/LICENSE.md</license>
// <author><NAME>, <EMAIL></author>
//-----------------------------------------------------------------------
namespace NSwag.CodeGeneration.ClientGenerators.TypeScript
{
/// <summary>Settings for the <see cref="SwaggerToTypeScriptGenerator"/>.</summary>
public class SwaggerToTypeScriptGeneratorSettings : ClientGeneratorBaseSettings
{
/// <summary>Initializes a new instance of the <see cref="SwaggerToTypeScriptGeneratorSettings"/> class.</summary>
public SwaggerToTypeScriptGeneratorSettings()
{
ModuleName = "";
ClassName = "{controller}Client";
Template = TypeScriptTemplate.JQueryCallbacks;
}
/// <summary>Gets or sets the class name of the service client.</summary>
public string ClassName { get; set; }
/// <summary>Gets or sets the output template.</summary>
public TypeScriptTemplate Template { get; set; }
/// <summary>Gets or sets the TypeScript module name (default: '', no module).</summary>
public string ModuleName { get; set; }
}
}<file_sep>/src/NSwagStudio/Views/ClientGenerators/CSharpClientGeneratorView.xaml.cs
using System.Threading.Tasks;
using System.Windows;
using MyToolkit.Mvvm;
using NSwag.CodeGeneration.ClientGenerators.CSharp;
using NSwag.Commands;
using NSwagStudio.ViewModels;
using NSwagStudio.ViewModels.ClientGenerators;
namespace NSwagStudio.Views.ClientGenerators
{
public partial class CSharpClientGeneratorView : IClientGenerator
{
public CSharpClientGeneratorView(SwaggerToCSharpCommand command)
{
InitializeComponent();
ViewModelHelper.RegisterViewModel(Model, this);
Model.Command = command;
}
public string Title { get { return "CSharp Client"; } }
private CSharpClientGeneratorViewModel Model { get { return (CSharpClientGeneratorViewModel) Resources["ViewModel"]; } }
public Task GenerateClientAsync(string swaggerData)
{
return Model.GenerateClientAsync(swaggerData);
}
}
}
<file_sep>/src/NSwag/Commands/SwaggerToTypeScriptCommand.cs
using System.ComponentModel;
using System.Threading.Tasks;
using NConsole;
using Newtonsoft.Json;
using NSwag.CodeGeneration.ClientGenerators;
using NSwag.CodeGeneration.ClientGenerators.TypeScript;
using NSwag.Commands.Base;
namespace NSwag.Commands
{
[Description("Generates TypeScript client code from a Swagger specification.")]
public class SwaggerToTypeScriptCommand : InputOutputCommandBase
{
public SwaggerToTypeScriptCommand()
{
Settings = new SwaggerToTypeScriptGeneratorSettings();
}
[JsonIgnore]
public SwaggerToTypeScriptGeneratorSettings Settings { get; set; }
[Description("The class name of the generated client.")]
[Argument(Name = "ClassName", DefaultValue = "{controller}Client")]
public string ClassName
{
get { return Settings.ClassName; }
set { Settings.ClassName = value; }
}
[Description("The TypeScript module name (default: '', no module).")]
[Argument(Name = "ModuleName", DefaultValue = "")]
public string ModuleName
{
get { return Settings.ModuleName; }
set { Settings.ModuleName = value; }
}
[Description("The type of the asynchronism handling ('JQueryCallbacks', 'JQueryQPromises', 'AngularJS').")]
[Argument(Name = "Template", DefaultValue = TypeScriptTemplate.JQueryCallbacks)]
public TypeScriptTemplate Template
{
get { return Settings.Template; }
set { Settings.Template = value; }
}
[Description("Specifies whether generate client classes.")]
[Argument(Name = "GenerateClientClasses", DefaultValue = true)]
public bool GenerateClientClasses
{
get { return Settings.GenerateClientClasses; }
set { Settings.GenerateClientClasses = value; }
}
[Description("Specifies whether generate interfaces for the client classes.")]
[Argument(Name = "GenerateClientInterfaces", DefaultValue = false)]
public bool GenerateClientInterfaces
{
get { return Settings.GenerateClientInterfaces; }
set { Settings.GenerateClientInterfaces = value; }
}
[Description("Specifies whether to generate DTO classes.")]
[Argument(Name = "GenerateDtoTypes", DefaultValue = true)]
public bool GenerateDtoTypes
{
get { return Settings.GenerateDtoTypes; }
set { Settings.GenerateDtoTypes = value; }
}
[Description("The operation generation mode ('SingleClientFromOperationId' or 'MultipleClientsFromPathSegments').")]
[Argument(Name = "OperationGenerationMode", DefaultValue = OperationGenerationMode.SingleClientFromOperationId)]
public OperationGenerationMode OperationGenerationMode
{
get { return Settings.OperationGenerationMode; }
set { Settings.OperationGenerationMode = value; }
}
public override async Task RunAsync(CommandLineProcessor processor, IConsoleHost host)
{
var output = await RunAsync();
WriteOutput(host, output);
}
public async Task<string> RunAsync()
{
var clientGenerator = new SwaggerToTypeScriptGenerator(InputSwaggerService, Settings);
return clientGenerator.GenerateFile();
}
}
} | 5a1c4d2c431e918328e86ba06f02120c06905fc2 | [
"C#"
] | 8 | C# | geekmi/NSwag | 7b48e199eb27563a584cdce184d68459641f30e0 | fc5cb330521a88c4467b2b6f3f70bdb09887325d | |
refs/heads/master | <repo_name>TheUltiOne/AFK<file_sep>/AFK/Handlers/AFKCommand.cs
using CommandSystem;
using Exiled.API.Features;
using RemoteAdmin;
using System;
using System.Linq;
namespace AFK
{
[CommandHandler(typeof(ClientCommandHandler))]
public class AFKCommand : ICommand
{
public string Command => "afk";
public string[] Aliases => Array.Empty<string>();
public string Description => "Gives a player an overwatch-like mode to prevent AFK kicking";
public bool Execute(ArraySegment<string> arguments, ICommandSender sender, out string response)
{
if (sender is PlayerCommandSender player)
{
if (Handlers.AFKHandlers.afk_players.Count() >= AFK.Instance.Config.AFKMaxplayers)
{
response = AFK.Instance.Config.AFKMaxPlayersMessage.Replace("%afkcount%", Convert.ToString(AFK.Instance.Config.AFKMaxplayers)); ;
return false;
}
Player p = Player.Get(player.SenderId);
if (Handlers.AFKHandlers.afk_players.Contains(p)) {
Handlers.AFKHandlers.afk_players.Remove(p);
} else
{
Handlers.AFKHandlers.afk_players.Add(p);
}
response = Handlers.AFKHandlers.afk_players.Contains(p)
? AFK.Instance.Config.AddedToAFK
: AFK.Instance.Config.RemovedFromAFK;
return true;
}
response = "This command must be executed from the game level.";
return false;
}
}
}
<file_sep>/README.md
# AFK
If command in the `~` console is .afk, you will go into overwatch mode.
This makes you __NOT__ respawn.
Running the command will take you out of overwatch mode.
IT SHOULD KILL YOU!
# Config Options
| Name | Type | Description | Default |
| --- | --- | --- | --- |
| `is_enabled` | bool | Toggles the plugin | true |
This plugin requires https://github.com/galaxy119/EXILED/releases/tag/2.1.18.
To install EXILED go to https://www.youtube.com/watch?v=EUfzj8OWvQU.
Once EXILED is installed, go to `appdata/roaming/exiled/plugins` and put it there. (windows)
LINUX: `.config/exiled/plugins`
Discord: TypicalIllusion#5726
<file_sep>/AFK/Config.cs
using System.ComponentModel;
using Exiled.API.Interfaces;
namespace AFK
{
public class Config : IConfig
{
[Description("Is the plugin enabled?")]
public bool IsEnabled { get; set; } = true;
[Description("How many players until the command doesnt work?")]
public int AFKMaxplayers { get; set; } = 3;
[Description("The response in console when removed from AFK.")]
public string RemovedFromAFK { get; set; } = "Removed from AFK!";
[Description("The response in console when added to AFK.")]
public string AddedToAFK { get; set; } = "Added to AFK!";
[Description("Sets the Hint content when the player is not affected by a respawn wave to let them know about their AFK mode.")]
public string AFKHintContent { get; set; } = "AFK Mode ON ~ To remove it, run .afk in the console. (~/`)";
[Description("Sets the Hint duration when the player is not affected by a respawn wave.")]
public float AFKHintDuration { get; set; } = 5;
[Description("Sets the response sent while trying to use the command but max players are AFK. (use %afkcount% for AFK max players)")]
public string AFKMaxPlayersMessage { get; set; } = "Max AFK players reached - %afkcount%/%afkcount%";
}
}
<file_sep>/AFK/AFK.cs
using Exiled.API.Features;
using Exiled.API.Enums;
using Server = Exiled.Events.Handlers.Server;
using Player = Exiled.Events.Handlers.Player;
using Map = Exiled.Events.Handlers.Map;
using System;
namespace AFK
{
public class AFK : Plugin<Config>
{
public override string Name { get; } = "AFKPlugin";
public override string Author { get; } = "TheUltiOne";
public override Version Version { get; } = new Version(1, 0, 0);
public override Version RequiredExiledVersion { get; } = new Version(2, 1, 29);
private static AFK Singleton = new AFK();
public override PluginPriority Priority { get; } = PluginPriority.Medium;
private Handlers.AFKHandlers handlers;
private AFK()
{
}
public static AFK Instance => Singleton;
public override void OnEnabled()
{
RegisterEvents();
}
public override void OnDisabled()
{
UnregisterEvents();
}
public override void OnReloaded()
{
UnregisterEvents();
RegisterEvents();
}
public void RegisterEvents()
{
handlers = new Handlers.AFKHandlers();
Server.RespawningTeam += handlers.OnRespawningTeam;
Server.RoundEnded += handlers.OnRoundEnded;
}
public void UnregisterEvents()
{
Server.RespawningTeam -= handlers.OnRespawningTeam;
Server.RoundEnded -= handlers.OnRoundEnded;
handlers = null;
}
}
}
<file_sep>/AFK/Handlers/AFKHandlers.cs
using System.Collections.Generic;
using System.Linq;
using Exiled.API.Features;
using Exiled.Events.EventArgs;
namespace AFK.Handlers
{
class AFKHandlers
{
public static List<Player> afk_players = new List<Player>();
public void OnRoundEnded(RoundEndedEventArgs ev) {
afk_players.Clear();
}
public void OnRespawningTeam(RespawningTeamEventArgs ev)
{
foreach (Player i in ev.Players.ToList())
{
if (afk_players.Contains(i))
{
ev.Players.Remove(i);
i.ShowHint(AFK.Instance.Config.AFKHintContent, AFK.Instance.Config.AFKHintDuration);
}
}
}
}
}
<file_sep>/AFK/Handlers/Server.cs
using System;
using Exiled.Events.EventArgs;
using EPlayer = Exiled.API.Features.Player;
using System.Collections.Generic;
namespace AFK.Handlers
{
class Server
{
public static List<EPlayer> afk_players = new List<EPlayer>();
public void OnRespawningTeam(RespawningTeamEventArgs ev)
{
foreach (EPlayer player in EPlayer.List)
{
if (afk_players.Contains(player))
{
ev.Players.Remove(player);
}
}
}
}
}
| 415df6579d2071859ccd0e730371f8fc7a2bc80d | [
"Markdown",
"C#"
] | 6 | C# | TheUltiOne/AFK | 5ca2ba0229ed7a371993aec7659832dd84c069a7 | a986d7a7f6c93cdcc254783bfd9cb84b3065e7fe | |
refs/heads/master | <file_sep>export { default as Button } from "./Button/index.vue";
export { default as H1 } from "./H1/index.vue";<file_sep>import { storiesOf } from '@storybook/html';
storiesOf('Views|Toolbar with list', module)
.add('Base', () => (`
<div class="panel pa-0">
<div class="toolbar toolbar--border py-3">
<div class="toolbar__section toolbar__section--fill">
<h3 class="h3 mb-0">Toolbar</h5>
</div>
<div class="toolbar__section">
<a class="button primary">Button</a>
</div>
</div>
<ul class="list list--divider">
<li class="list__item">
<a href="#" class="list__anchor">
<div class="list__column">
<h4 class="h5">Title 1</h4>
<p class="paragraph mb-0">Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.</p>
</div>
</a>
</li>
<li class="list__item">
<a href="#" class="list__anchor">
<div class="list__column">
<h4 class="h5">Title 2</h4>
<p class="paragraph mb-0">Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.</p>
</div>
</a>
</li>
</ul>
</div>
`))<file_sep>import { storiesOf } from '@storybook/html';
storiesOf('Components|Toolbar', module)
.add('Base', () => (`
<div class="toolbar toolbar--box-shadow py-3">
<h5 class="h5 mb-0">Toolbar</h5>
</div>
`))
.add('Center', () => (`
<div class="toolbar toolbar--box-shadow py-3">
<div class="toolbar__section toolbar__section--centered">
<h5 class="h5 mb-0">Toolbar</h5>
</div>
</div>
`))
// .add('Sections', () => (`
// <div class="toolbar toolbar--box-shadow py-3">
// <div class="toolbar__left-section">
// <a class="button primary mx-3">Button</a>
// </div>
// <div class="toolbar__left-section">
// <strong class="mx-3">Toolbar</strong>
// </div>
// <div class="toolbar__right-section">
// <a class="button primary mx-3">Button</a>
// </div>
// </div>
// `))
.add('Sections', () => (`
<div class="toolbar toolbar--box-shadow py-3">
<div class="toolbar__section toolbar__section--fill">
<h5 class="h5 mb-0">Toolbar</h5>
</div>
<div class="toolbar__section">
<a class="button primary">Button</a>
</div>
</div>
`))
.add('Orientation', () => (`
<div class="panel pa-0 mb-5">
<div class="toolbar toolbar--border py-3">
<h5 class="h5 mb-0">Top</h5>
</div>
<div class="pa-3">
Hello World
</div>
</div>
<div class="panel pa-0">
<div class="pa-3">
Hello World
</div>
<div class="toolbar toolbar--border toolbar--bottom py-3">
<h5 class="h5 mb-0">Bottom</h5>
</div>
</div>
`))<file_sep>import Vue from 'vue';
import { storiesOf } from '@storybook/vue';
import { Button } from "@components/vue";
Vue.component("v-button", Button);
storiesOf('Button', module)
.add('Base', () => ({
template: `<v-button>Button</v-button>`
}))
.add('Variant', () => ({
template: `
<div>
<v-button variant="primary">Primary</v-button>
<v-button variant="secondary">Secondary</v-button>
<v-button variant="success">Success</v-button>
<v-button variant="danger">Danger</v-button>
</div>
`
}))
.add('Size', () => ({
template: `
<div>
<v-button size="small">Small</v-button>
<v-button>Regular</v-button>
<v-button size="large">Large</v-button>
</div>
`
}))<file_sep>import { storiesOf } from '@storybook/html';
storiesOf('Base|Font-size', module)
.add('All', () => (`
<p class="fs-1 my-1">Hello World</p>
<p class="fs-2 my-1">Hello World</p>
<p class="fs-3 my-1">Hello World</p>
<p class="fs-4 my-1">Hello World</p>
<p class="fs-5 my-1">Hello World</p>
<p class="fs-6 my-1">Hello World</p>
<p class="fs-7 my-1">Hello World</p>
<p class="fs-8 my-1">Hello World</p>
<p class="fs-9 my-1">Hello World</p>
<p class="fs-10 my-1">Hello World</p>
<p class="fs-11 my-1">Hello World</p>
<p class="fs-12 my-1">Hello World</p>
<p class="fs-13 my-1">Hello World</p>
<p class="fs-14 my-1">Hello World</p>
<p class="fs-15 my-1">Hello World</p>
<p class="fs-16 my-1">Hello World</p>
`))<file_sep>import { storiesOf } from '@storybook/html';
storiesOf('Components|Heading', module)
.add('All', () => (`
<h1 class="h1">Hello World</h1>
<p class="paragraph mb-8">Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.</p>
<h2 class="h2">Hello World</h2>
<p class="paragraph mb-8">Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.</p>
<h3 class="h3">Hello World</h3>
<p class="paragraph mb-8">Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.</p>
<h4 class="h4">Hello World</h4>
<p class="paragraph mb-8">Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.</p>
<h5 class="h5">Hello World</h5>
<p class="paragraph mb-8">Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.</p>
<h6 class="h6">Hello World</h6>
<p class="paragraph mb-8">Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.</p>
`))<file_sep>module.exports = ({ config }) => {
config.module.rules.push({
test: /\.(ts|tsx)$/,
loader: "ts-loader"
});
config.resolve.extensions.push(".ts", ".tsx");
config.module.rules.push({
test: /\.scss$/,
use: [
'style-loader',
'css-loader',
'sass-loader'
]
});
return config;
};
// {
// loader: 'css-loader',
// options: {
// modules: {
// mode: 'local',
// localIdentName: '[local]-[hash:base64:5]',
// },
// importLoaders: 1
// },
// },<file_sep>import { storiesOf } from '@storybook/html';
storiesOf('Components|Button', module)
.add('Base', () => (`
<a class="button">Button</a>
`))
.add('Variant', () => (`
<a class="button button--primary">Primary</a>
<a class="button button--secondary">Secondary</a>
<a class="button button--success">Success</a>
<a class="button button--danger">Danger</a>
`))
.add('Size', () => (`
<a class="button button--small">Small</a>
<a class="button">Regular</a>
<a class="button button--large">Large</a>
`))<file_sep>import { storiesOf } from '@storybook/html';
storiesOf('Components|List', module)
.add('Base', () => (`
<ul class="list">
<li class="list__item">Hello</li>
<li class="list__item">World</li>
</ul>
`))
.add('Divider', () => (`
<ul class="list list--divider">
<li class="list__item">Hello</li>
<li class="list__item">World</li>
</ul>
`))
.add('Anchor', () => (`
<ul class="callout list list--divider">
<li class="list__item">
<a href="#" class="list__anchor">Hello</a>
</li>
<li class="list__item">
<a href="#" class="list__anchor">World</a>
</li>
</ul>
`))
.add('Columns', () => (`
<ul class="callout list list--divider">
<li class="list__item">
<div class="list__column list__column--fill">
<h4 class="h4">Title 1</h4>
<p class="paragraph mb-1">Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.</p>
</div>
<div class="list__column">
<a class="button primary mx-3">Button</a>
</div>
</li>
<li class="list__item">
<a href="#" class="list__anchor">
<div class="list__column">
<h4 class="h4">Title 2</h4>
<p class="paragraph mb-1">Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.</p>
</div>
</a>
</li>
</ul>
`))<file_sep>import { storiesOf } from '@storybook/html';
storiesOf('Components|Callout', module)
.add('Base', () => (`
<div class="callout">Hello World</div>
`))<file_sep>import Vue from 'vue';
import { storiesOf } from '@storybook/vue';
import { H1 } from "@components/vue";
Vue.component("v-h1", H1);
storiesOf('H1', module)
.add('Base', () => ({
template: `<v-h1>H1</v-h1>`
}))<file_sep>import { storiesOf } from '@storybook/html';
storiesOf('Components|Panel', module)
.add('Base', () => (`
<div class="panel">Hello World</div>
`))<file_sep>module.exports = ({ config }) => {
config.module.rules.push({
test: /\.(ts|tsx)$/,
loader: "ts-loader",
options: { appendTsSuffixTo: [/\.vue$/] }
});
config.resolve.extensions.push(".ts", ".tsx");
config.module.rules.push({
test: /\.scss$/,
use: [
'vue-style-loader',
'css-loader',
'sass-loader'
]
});
return config;
};<file_sep>import { storiesOf } from '@storybook/html';
storiesOf('Components|UL', module)
.add('Base', () => (`
<ul class="ul">
<li>Hello</li>
<li>World</li>
</ul>
`))
.add('Compact', () => (`
<ul class="ul ul--compact">
<li>Hello</li>
<li>World</li>
</ul>
`)) | 4c83c6d9756581b73a341bd2c4082fdc2f9bb249 | [
"JavaScript",
"TypeScript"
] | 14 | TypeScript | mwildeboer/design-system | 941cb47fabb77d5348ec793b0266ea328e51ebd2 | d6768d7f176134126c6ee0e90a7a7690100d41bb | |
refs/heads/master | <file_sep>FROM postgres:latest
LABEL maintainer="<NAME>"
<file_sep># Generated by Django 3.0.7 on 2021-01-13 16:54
import datetime
from django.db import migrations, models
class Migration(migrations.Migration):
dependencies = [
('chronicles_backend', '0001_initial'),
]
operations = [
migrations.AlterField(
model_name='bugreport',
name='creation',
field=models.DateTimeField(default=datetime.datetime.now, verbose_name='Timestamp of bug report'),
),
migrations.AlterField(
model_name='comment',
name='creation',
field=models.DateTimeField(default=datetime.datetime.now, verbose_name='Timestamp of comment'),
),
migrations.AlterField(
model_name='project',
name='creation',
field=models.DateTimeField(default=datetime.datetime.now, verbose_name='Timestamp of project creation'),
),
]
<file_sep>aioredis==1.3.1
appdirs==1.4.3
asgiref==3.2.7
async-timeout==3.0.1
attrs==19.3.0
autobahn==20.12.3
Automat==20.2.0
CacheControl==0.12.6
certifi==2020.4.5.1
cffi==1.14.0
channels==2.4.0
channels-redis==2.4.2
chardet==3.0.4
colorama==0.4.3
constantly==15.1.0
contextlib2==0.6.0
cryptography==3.3.2
daphne==2.5.0
distlib==0.3.0
distro==1.4.0
Django==3.1.12
django-cors-headers==3.2.1
django-environ==0.4.5
django-model-utils==4.0.0
djangorestframework==3.11.2
gunicorn==20.0.4
hiredis==1.0.1
html5lib==1.0.1
hyperlink==19.0.0
idna==2.9
incremental==17.5.0
ipaddr==2.2.0
lockfile==0.12.2
msgpack==0.6.2
packaging==20.3
pep517==0.8.2
Pillow==8.2.0
progress==1.5
psycopg2==2.8.6
pyasn1==0.4.8
pyasn1-modules==0.2.8
pycparser==2.20
PyHamcrest==2.0.2
pyOpenSSL==19.1.0
pyparsing==2.4.6
python-decouple==3.3
pytoml==0.1.21
pytz==2020.1
PyYAML==5.4
requests==2.23.0
retrying==1.3.3
service-identity==18.1.0
six==1.15.0
sqlparse==0.3.1
Twisted==20.3.0
txaio==20.4.1
urllib3==1.26.5
webencodings==0.5.1
zope.interface==5.1.0
<file_sep>import json
from asgiref.sync import async_to_sync
from channels.db import database_sync_to_async
from channels.generic.websocket import WebsocketConsumer
from channels.auth import get_user
from .models import BugReport, Comment
from .serializers import CommentVerboseSerializer
class CommentConsumer(WebsocketConsumer):
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.bug_id = self.scope['url_route']['kwargs']['pk']
def connect(self):
self.user = async_to_sync(get_user)(self.scope)
if self.user.is_authenticated:
try:
bug_report = BugReport.objects.get(pk=self.bug_id)
async_to_sync(self.channel_layer.group_add)(
self.bug_id,
self.channel_name
)
self.accept()
except BugReport.DoesNotExist:
self.close()
else:
self.close()
def disconnect(self, code):
async_to_sync(self.channel_layer.group_discard)(
self.bug_id,
self.channel_name
)
def receive(self, text_data=None, bytes_data=None):
text_data_json = json.loads(text_data)
comment_id = text_data_json['comment_id']
try:
comment = Comment.objects.get(pk=comment_id)
comment_serializer = CommentVerboseSerializer(comment)
async_to_sync(self.channel_layer.group_send)(
self.bug_id,
{
'type': 'send_comment',
'comment': comment_serializer.data,
}
)
except Comment.DoesNotExist:
pass
def send_comment(self, event):
comment = event['comment']
self.send(text_data=json.dumps(comment))
<file_sep>from rest_framework import serializers
from chronicles_backend.models import *
class UserSerializer(serializers.ModelSerializer):
class Meta:
model = ChronicleUser
fields = ['id', 'username', 'first_name', 'last_name', 'isAdmin', 'is_active']
class ProjectSerializer(serializers.ModelSerializer):
class Meta:
model = Project
fields = ['id', 'name', 'description', 'creator', 'team', 'creation', 'image', 'slug']
read_only_fields = ['creator', 'creation']
lookup_field = 'slug'
extra_kwargs = {
'url': {'lookup_field': 'slug'}
}
class BugReportSerializer(serializers.ModelSerializer):
class Meta:
model = BugReport
fields = ['id', 'project', 'reporter', 'heading', 'description',
'person_in_charge', 'creation', 'status', 'tagsHash']
read_only_fields = ['reporter', 'creation', 'status', 'person_in_charge']
class BugReportEditSerializer(serializers.ModelSerializer):
class Meta:
model = BugReport
fields = ['id', 'project', 'reporter', 'heading', 'description',
'person_in_charge', 'creation', 'status', 'tagsHash']
read_only_fields = ['reporter', 'creation', 'project', 'heading', 'description', 'tagsHash']
class BugReportVerboseSerializer(serializers.ModelSerializer):
reporter = UserSerializer()
person_in_charge = UserSerializer()
project = ProjectSerializer()
class Meta:
model = BugReport
fields = ['id', 'project', 'reporter', 'heading', 'description',
'person_in_charge', 'creation', 'status', 'tagsHash']
read_only_fields = ['reporter', 'creation', 'project', 'heading', 'description', 'tagsHash']
class CommentSerializer(serializers.ModelSerializer):
class Meta:
model = Comment
fields = ['id', 'report', 'creation', 'commenter', 'body']
read_only_fields = ['commenter', 'creation']
class CommentEditSerializer(serializers.ModelSerializer):
class Meta:
model = Comment
fields = ['id', 'report', 'creation', 'commenter', 'body']
read_only_fields = ['commenter', 'creation', 'report']
class CommentVerboseSerializer(serializers.ModelSerializer):
commenter = UserSerializer()
class Meta:
model = Comment
fields = ['id', 'report', 'creation', 'commenter', 'body']
read_only_fields = ['commenter', 'creation']
class ImageSerializer(serializers.ModelSerializer):
class Meta:
model = Image
fields = ['id', 'randIdentifier', 'url']
# Tags legend:
# 0 :Functionality
# 1 :Usability
# 2 :Interface
# 3 :Compatibility
# 4 :Performance
# 5 :Security
# 6 :Management
# 7 :Code
# 8 :UI
# 9 :UX
# 10:New Feature
# 11:Fat gaya
# 12:Design
# 13:Front end
# 14:Back end
# 15:Database
# 16:External Resource
# 17:Coverage
# 18:Bug
# 19:Improvement
# 20:Broken
# 21:Cookie
# 22:Typo
# 23:
<file_sep>FROM python:3.8.5-alpine
LABEL maintainer="<NAME>"
ENV PYTHONUNBUFFERED 1
RUN apk update && apk add postgresql-dev gcc python3-dev musl-dev libffi-dev openssl-dev jpeg-dev zlib-dev libjpeg
RUN mkdir /code
WORKDIR /code
COPY requirements.txt /code/
RUN pip install --upgrade pip
RUN pip install -r requirements.txt
COPY . .
<file_sep>from django.db import models
from django.contrib.auth.models import AbstractUser
from datetime import datetime
class ChronicleUser(AbstractUser):
enrNo = models.IntegerField(default=0)
isAdmin = models.BooleanField(default=False)
is_active = models.BooleanField(default=True)
class Meta:
ordering = ['enrNo']
def __str__(self):
return self.get_username()
class Project(models.Model):
name = models.CharField(max_length=100)
description = models.CharField(max_length=10000)
creator = models.ForeignKey(ChronicleUser, null=True, on_delete=models.SET_NULL, related_name='created_projects')
team = models.ManyToManyField(ChronicleUser, related_name='projects')
creation = models.DateTimeField(default=datetime.now, verbose_name='Timestamp of project creation')
image = models.ImageField(upload_to='projectImages/', default='defaults/ProjectLogo.png')
slug = models.CharField(max_length=100, unique=True)
def __str__(self):
return self.name
class BugReport(models.Model):
project = models.ForeignKey(Project, on_delete=models.CASCADE)
reporter = models.ForeignKey(ChronicleUser, null=True, on_delete=models.SET_NULL, related_name='reported_bugs')
heading = models.CharField(max_length=500)
description = models.CharField(max_length=15000)
person_in_charge = models.ForeignKey(ChronicleUser, null=True, on_delete=models.SET_NULL,
related_name='bugs_assigned')
creation = models.DateTimeField(default=datetime.now, verbose_name='Timestamp of bug report')
status = models.BooleanField(default=False)
tagsHash = models.IntegerField(default=0)
class Meta:
ordering = ['status', 'creation']
def __str__(self):
return f"{self.project}::{self.heading}"
class Comment(models.Model):
report = models.ForeignKey(BugReport, on_delete=models.CASCADE)
creation = models.DateTimeField(default=datetime.now, verbose_name='Timestamp of comment')
commenter = models.ForeignKey(ChronicleUser, null=True, on_delete=models.SET_NULL)
body = models.CharField(max_length=1000)
class Meta:
ordering = ['creation']
def __str__(self):
return f"{self.report.project}::{self.report}::{self.body}"
class Image(models.Model):
randIdentifier = models.CharField(max_length=100)
url = models.ImageField(upload_to='imageWarehouse/')
<file_sep>from django.urls import re_path
from . import consumers
websocket_urlpatterns = [
re_path(r'ws/bugReport/(?P<pk>\d+)/$', consumers.CommentConsumer)
]
<file_sep>FROM redis:5
LABEL maintainer="<NAME>"
<file_sep>from chronicles.settings import CONFIG_VARS
import json
import requests
from django.contrib.auth import login, logout
from django.http import JsonResponse, HttpResponseForbidden, HttpResponseBadRequest
from rest_framework import viewsets
from rest_framework.decorators import action
from rest_framework.response import Response
from rest_framework.views import APIView
from .permissions import *
from .serializers import *
from .mailingWizard import MailThread
class UserViewSet(viewsets.ModelViewSet):
queryset = ChronicleUser.objects.all()
serializer_class = UserSerializer
permission_classes = [IsAdmin]
@action(methods=['GET'], detail=False, url_path='projects', url_name='projects')
def user_projects(self, request):
if request.user.is_authenticated and request.user.is_active:
serializer = ProjectSerializer(request.user.projects.all(), many=True)
return Response(serializer.data)
else:
return HttpResponseForbidden()
@action(methods=['GET'], detail=False, url_path='bugReports', url_name='bugReports')
def user_bug_reports(self, request):
if request.user.is_authenticated and request.user.is_active:
serializer = BugReportVerboseSerializer(request.user.bugs_assigned.all(), many=True)
return Response(serializer.data)
else:
return HttpResponseForbidden()
@action(methods=['GET'], detail=False, url_path='curr', url_name='curr')
def curr_user(self, request):
if request.user.is_authenticated:
user = request.user
if user.is_active:
serializer = UserSerializer(user)
return JsonResponse(serializer.data)
else:
return HttpResponseForbidden()
else:
return HttpResponseForbidden()
@action(methods=['GET'], detail=False, url_path='logout', url_name='logout')
def logoutCU(self, request):
if request.user.is_authenticated:
logout(request)
return JsonResponse({'status': 'Logged out'})
else:
return HttpResponseForbidden()
@action(methods=['POST', 'OPTIONS'], detail=False, url_name='token', url_path='token')
def token_parser(self, request):
try:
data = json.loads(request.body.decode('utf-8'))
auth_code = data['code']
except:
return HttpResponseBadRequest()
payload = {
'client_id': CONFIG_VARS['CHANNELI']['CLIENT_ID'],
'client_secret': CONFIG_VARS['CHANNELI']['CLIENT_SECRET'],
'grant_type': 'authorization_code',
'redirect_url': CONFIG_VARS['CHANNELI']['REDIRECT_URI'],
'code': auth_code,
}
response = requests.post('https://internet.channeli.in/open_auth/token/', data=payload)
resdict = json.loads(response.text)
access_token = resdict['access_token']
response2 = requests.get("https://internet.channeli.in/open_auth/get_user_data/",
headers={'Authorization': f'Bearer {access_token}'})
resdict2 = json.loads(response2.text)
# Login
roles = resdict2['person']['roles']
maintainer = False
for i in roles:
if i['role'] == 'Maintainer':
maintainer = True
if maintainer:
try:
user = ChronicleUser.objects.get(enrNo=resdict2['student']['enrolmentNumber'])
if user.is_active:
login(request=request, user=user)
else:
return HttpResponseForbidden()
except ChronicleUser.DoesNotExist:
enr_no = resdict2['student']['enrolmentNumber']
if (int(enr_no) // (10 ** 6)) % 10 == 7:
staff = True
else:
staff = False
user = ChronicleUser(
username=resdict2['person']['fullName'],
enrNo=resdict2['student']['enrolmentNumber'],
email=resdict2['contactInformation']['instituteWebmailAddress'],
is_staff=staff,
isAdmin=staff,
is_superuser=staff
)
user.save()
login(request=request, user=user)
return JsonResponse({'user': user.username})
class ProjectViewSet(viewsets.ModelViewSet):
queryset = Project.objects.all()
serializer_class = ProjectSerializer
lookup_field = 'slug'
permission_classes = [permissions.IsAuthenticated, IsProjectCreatorOrAdmin]
def perform_create(self, serializer):
project = serializer.save(creator=self.request.user)
context = {
"action": "new_team_member",
"project": project,
}
MailThread(serializer.validated_data['team'], context).start()
def perform_update(self, serializer):
try:
new_users = [user for user in serializer.validated_data['team'] if user not in self.get_object().team.all()]
context = {
"action": "new_team_member",
"project": self.get_object(),
}
MailThread(new_users, context).start()
except KeyError:
pass
serializer.save()
class BugReportViewSet(viewsets.ModelViewSet):
queryset = BugReport.objects.all()
permission_classes = [permissions.IsAuthenticated, IsTeamMemberOrAdmin]
def perform_create(self, serializer):
bug_report = serializer.save(reporter=self.request.user)
context = {
"action": "bug_report_update",
"project": bug_report.project,
"bug_report": bug_report,
}
MailThread(bug_report.project.team.all(), context).start()
def perform_update(self, serializer):
try:
user = serializer.validated_data['person_in_charge']
status = serializer.validated_data['status']
if status:
context = {
"action": "bug_resolved",
"project": self.get_object().project,
"bug_report": self.get_object(),
}
MailThread(self.get_object().project.team.all(), context).start()
else:
context = {
"action": "bug_report_assignment",
"project": self.get_object().project,
"bug_report": self.get_object(),
}
MailThread([user], context).start()
except KeyError:
pass
serializer.save()
def get_serializer_class(self):
if self.request.method == 'POST':
return BugReportSerializer
elif self.request.method == 'PATCH' or self.request.method == 'PUT':
return BugReportEditSerializer
else:
return BugReportVerboseSerializer
def partial_update(self, request, *args, **kwargs):
kwargs['partial'] = True
if ChronicleUser.objects.get(pk=request.data['person_in_charge']) in self.get_object().project.team.all():
return self.update(request, *args, **kwargs)
else:
content = {
'status': 'Not a team member'
}
return Response(content)
def update(self, request, *args, **kwargs):
if ChronicleUser.objects.get(pk=request.data['person_in_charge']) in self.get_object().project.team.all():
partial = kwargs.pop('partial', False)
instance = self.get_object()
serializer = self.get_serializer(instance, data=request.data, partial=partial)
serializer.is_valid(raise_exception=True)
self.perform_update(serializer)
return Response(serializer.data)
else:
content = {
'status': 'Not a team member'
}
return Response(content)
class CommentViewSet(viewsets.ModelViewSet):
queryset = Comment.objects.all()
permission_classes = [permissions.IsAuthenticated, IsCommenter]
def perform_create(self, serializer):
serializer.save(commenter=self.request.user)
def get_serializer_class(self):
if self.request.method == 'POST':
return CommentSerializer
else:
return CommentEditSerializer
class MembersOfProject(APIView):
def get(self, request, pk, format=None):
serializer = UserSerializer(Project.objects.get(pk=pk).team.all(), many=True)
return Response(serializer.data)
class BugsOfProject(APIView):
def get(self, request, pk, format=None):
serializer = BugReportSerializer(Project.objects.get(pk=pk).bugreport_set.all(), many=True)
return Response(serializer.data)
class CommentsOnBugs(APIView):
def get(self, request, pk, format=None):
serializer = CommentVerboseSerializer(BugReport.objects.get(pk=pk).comment_set.all(), many=True)
return Response(serializer.data)
class ImageViewSet(viewsets.ModelViewSet):
queryset = Image.objects.all()
serializer_class = ImageSerializer
permission_classes = [permissions.IsAuthenticated]
@action(methods=['POST'], detail=False, url_path='deleteRem', url_name='deleteRem')
def delete_remaining_images(self, request):
if request.user.is_authenticated:
# try:
randIdentifier = request.POST.get('randIdentifier')
urls = request.POST.get('urls')
query_set = Image.objects.filter(randIdentifier=randIdentifier)
for i in query_set:
if i.url.url not in urls:
i.delete()
return JsonResponse({'status': 'successful'})
# except:
# return HttpResponseBadRequest()
else:
return HttpResponseForbidden()
<file_sep>FROM nginx:alpine
LABEL maintainer="<NAME>"
RUN rm /etc/nginx/conf.d/default.conf
COPY ./configuration/ /etc/nginx/conf.d/
<file_sep>from chronicles.settings import CONFIG_VARS
import smtplib, ssl
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
import threading
import io
import yaml
with io.open('configuration/config.yml', 'r') as stream:
CONFIG_VARS = yaml.safe_load(stream)
sender_email = CONFIG_VARS['EMAIL']['ADDRESS']
password = CONFIG_VARS['EMAIL']['PASSWORD']
def sendmail(receiver, message):
context = ssl.create_default_context()
with smtplib.SMTP_SSL("smtp.gmail.com", 465, context=context) as server:
server.login(sender_email, password)
server.sendmail(sender_email, receiver, message.as_string())
def message_generator(mail_data):
message = MIMEMultipart("alternative")
message["Subject"] = mail_data["subject"]
message["From"] = sender_email
message["To"] = mail_data["receiver_email"]
text = mail_data["text"]
html = mail_data["html"]
part1 = MIMEText(text, "plain")
part2 = MIMEText(html, "html")
message.attach(part1)
message.attach(part2)
return message
def personify(user_list, context):
frontend_url = CONFIG_VARS['FRONTEND']
subject = ""
text = ""
html = ""
if context["action"] == "new_team_member":
subject = "Welcome Aboard!"
text = f"""You have been added to the project {context['project'].name}"""
html = f"""\
<html>
<body style='text-align:center;'>
<h1 style='color:purple;'>Chronicles</h1><hr />
<p>Congratulations on making into Project <strong>{context['project'].name}</strong>!</p><hr />
</body>
</html>
"""
elif context["action"] == "bug_report_update":
subject = f"New Bug found in {context['project'].name} 😟"
text = f"""There is a new bug report in {context['project'].name}.
Visit {frontend_url+context['project'].slug}/
"""
html = f"""\
<html>
<body style='text-align:center;'>
<h1 style='color:purple;'>Chronicles</h1><hr />
<p>Project <strong>{context['project'].name}</strong> has a new bug report
entitled {context['bug_report'].heading}.</p>
<p>Visit Chronicles and fix it ASAP!</p>
<a href="{frontend_url+context['project'].slug}/">Link to the Project</a><hr />
</body>
</html>
"""
elif context["action"] == "bug_report_assignment":
subject = f"Bug report assigned to you!"
text = f"""A bug of project {context['project'].name} has been assigned to you.
Visit {frontend_url} to find out more.
"""
html = f"""\
<html>
<body style='text-align:center;'>
<h1 style='color:purple;'>Chronicles</h1><hr />
<p>A bug in the project <strong>{context['project'].name}</strong> has been assigned to you.</p>
<p>Heading: {context['bug_report'].heading}</p>
<p>Visit Chronicles and fix it ASAP!</p>
<a style="color:'purple'; text-decoration: 'none'" href="{frontend_url}/">Chronicles</a><hr />
</body>
</html>
"""
elif context["action"] == "bug_resolved":
subject = f"Bug report resolved 😊"
text = f"""A bug of project {context['project'].name} has been resolved!"""
html = f"""\
<html>
<body style='text-align:center;'>
<h1 style='color:purple;'>Chronicles</h1><hr />
<h3>Wohoo!</h3>
<p>A bug report from project <strong>{context['project'].name}</strong> was resolved!</p>
<h4>Heading of bug report:</h4>
<p>{context['bug_report'].heading}</p><hr />
</body>
</html>
"""
if (subject != "") and (text != "") and (html != ""):
for user in user_list:
mail_data = {
"receiver_email": user.email,
"subject": subject,
"text": text,
"html": html,
}
message = message_generator(mail_data)
print("Sending email")
sendmail(user.email, message)
print("Mail sent")
else:
print("No data")
class MailThread(threading.Thread):
def __init__(self, user_list, context, *args, **kwargs):
self.user_list = user_list
self.context = context
super(MailThread, self).__init__(*args, **kwargs)
def run(self):
personify(self.user_list, self.context)
return
<file_sep>from django.urls import path, include
from rest_framework.routers import DefaultRouter
from chronicles_backend.views import *
from django.conf.urls import include
router = DefaultRouter()
router.register(r'users', UserViewSet)
router.register(r'projects', ProjectViewSet)
router.register(r'bugReports', BugReportViewSet)
router.register(r'comments', CommentViewSet)
router.register(r'images', ImageViewSet)
urlpatterns = [
path('', include(router.urls)),
path('projects/<int:pk>/team/', MembersOfProject.as_view()),
path('projects/<int:pk>/bugReports/', BugsOfProject.as_view()),
path('bugReports/<int:pk>/comments/', CommentsOnBugs.as_view()),
]
<file_sep>from django.contrib import admin
from .models import *
admin.site.register(ChronicleUser)
admin.site.register(Project)
admin.site.register(BugReport)
admin.site.register(Comment)
admin.site.register(Image)
<file_sep>from rest_framework import permissions
# Permissions for Project View
class IsProjectCreatorOrAdmin(permissions.BasePermission):
def has_object_permission(self, request, view, obj):
if (request.method in permissions.SAFE_METHODS) or (request.method == 'POST'):
return True
return (obj.creator == request.user) or request.user.isAdmin
class IsTeamMemberOrAdmin(permissions.BasePermission):
def has_object_permission(self, request, view, obj):
if (request.method == 'POST') or (request.method in permissions.SAFE_METHODS):
return True
if request.user.isAdmin or (request.user in obj.project.team.all()):
return True
return False
class IsCommenter(permissions.BasePermission):
def has_object_permission(self, request, view, obj):
if (request.method in permissions.SAFE_METHODS) or (request.method == 'POST'):
return True
return request.user == obj.commenter
class IsAdmin(permissions.BasePermission):
def has_object_permission(self, request, view, obj):
if (request.method in permissions.SAFE_METHODS) or request.user.isAdmin:
return True
return False
<file_sep># Chronicles
This repository houses code for **Chronicles**, a platform where debuggers and developers unite to exterminate bugs. You are looking at the backend of the project with all the files required for dockerising it's implementation. Do visit the [Frontend](https://github.com/aitalshashank2/Chronicles-Frontend) of this project.
## Features
- Create Multiple Projects with a unique team.
- Secure Login and Registration using OAuth2.0.
- Report bugs in a project and use various in-built tags to highlight them.
- Implementation of RichText Fields for descriptions of Project and Bugs.
- Option to upload images in the description for Bug Reports.
- Real-time commenting on Bug Reports using web sockets
- Option to assign a bug report to a team member.
- Show the status of Bug Report
- Look at your Projects and the Bugs Reports assigned to you, all in one place
## Set up instructions
- Clone this repository and change directory
```bash
git clone https://github.com/aitalshashank2/Chronicles.git
cd Chronicles
```
- Clone the Frontend Web Application inside the `frontend/` directory
```bash
cd frontend/
git clone https://github.com/aitalshashank2/Chronicles-Frontend.git
```
- Copy `code/configuration/config-stencil.yml` to `code/configuration/config.yml` and populate the values.
```bash
cd ../code/configuration/
cp config-stencil.yml config.yml
```
- Build the project after returning to the base directory (containing the `docker-compose.yml` file)
```bash
docker-compose build
```
- Run the project
```bash
docker-compose up -d
```
- Visit **Chronicles** on [http://localhost:54330/](http://localhost:54330/)
- In order to stop the project, navigate to the base directory (containing the `docker-compose.yml` file) and run the following command
```bash
docker-compose down
```
### Happy Coding!
<file_sep>FROM node:14.4.0-alpine3.10
LABEL maintainer="<NAME>"
USER root
RUN mkdir /frontend
WORKDIR /frontend
COPY ./Chronicles-Frontend/ .
RUN npm install
RUN npm run build
| d9aa9e7f9e67b378c65f3e5dc4bf884614edf78e | [
"Markdown",
"Python",
"Text",
"Dockerfile"
] | 17 | Dockerfile | aitalshashank2/Chronicles | 82204bc75bf4afbc4f97e78e5636e6d88b3ae7bc | 07bc938c911b39dd5e4f33ebaa35fca356e1935c | |
refs/heads/master | <file_sep>#!/bin/bash
# Author: yeho <lj2007331 AT gmail.com>
# BLOG: https://blog.linuxeye.cn
#
# Notes: OneinStack for CentOS/RadHat 6+ Debian 6+ and Ubuntu 12+
#
# Project home page:
# https://oneinstack.com
# https://github.com/lj2007331/oneinstack
# check MySQL dir
[ -d "$mysql_install_dir/support-files" ] && { db_install_dir=$mysql_install_dir; db_data_dir=${mysql_data_dir}; }
[ -d "${mariadb_install_dir}/support-files" ] && { db_install_dir=${mariadb_install_dir}; db_data_dir=${mariadb_data_dir}; }
[ -d "${percona_install_dir}/support-files" ] && { db_install_dir=${percona_install_dir}; db_data_dir=${percona_data_dir}; }
[ -d "$alisql_install_dir/support-files" ] && { db_install_dir=$alisql_install_dir; db_data_dir=$alisql_data_dir; }
# check Nginx dir
[ -e "$nginx_install_dir/sbin/nginx" ] && web_install_dir=$nginx_install_dir
[ -e "$tengine_install_dir/sbin/nginx" ] && web_install_dir=$tengine_install_dir
[ -e "$openresty_install_dir/nginx/sbin/nginx" ] && web_install_dir=$openresty_install_dir/nginx
<file_sep>#!/bin/bash
# Author: yeho <lj2007331 AT gmail.com>
# BLOG: https://blog.linuxeye.com
#
# Notes: OneinStack for CentOS/RadHat 5+ Debian 6+ and Ubuntu 12+
#
# Project home page:
# http://oneinstack.com
# https://github.com/lj2007331/oneinstack
[ -e "$nginx_install_dir/sbin/nginx" ] && web_install_dir=$nginx_install_dir
[ -e "$tengine_install_dir/sbin/nginx" ] && web_install_dir=$tengine_install_dir
[ -e "$openresty_install_dir/nginx/sbin/nginx" ] && web_install_dir=$openresty_install_dir/nginx
| 69d6967bc7e4b6c62b1221e8caa4af17a1cca98a | [
"Shell"
] | 2 | Shell | apeny/lnmp | eba29c4c2dcb92060bcaf3b57fbf0d1b8b29f6b9 | 1a5db31f70f9f90fc9e1d4a2d129cd169f10b703 | |
refs/heads/master | <repo_name>nguyenthaison/bookinghotel<file_sep>/storage/framework/views/dcdb584f0ffaa671d40225355e752bd7c7d3b130.php
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1, user-scalable=no">
<title><?php echo $__env->yieldContent('title'); ?></title>
<!-- FONT -->
<link href='https://fonts.googleapis.com/css?family=Biryani:400,200,300,700' rel='stylesheet' type='text/css'>
<link href='https://fonts.googleapis.com/css?family=Lato:400,100,300,700' rel='stylesheet' type='text/css'>
<link rel="stylesheet" href="<?php echo e(url("assets/css/font-awesome.min.css")); ?>">
<!-- Latest compiled and minified CSS -->
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" integrity="<KEY>" crossorigin="anonymous">
<!-- Optional theme -->
<!-- CSS -->
<link rel="stylesheet" type="text/css" href="<?php echo e(url("/assets/css/style.css")); ?>">
<link rel="stylesheet" href="<?php echo e(url("/assets/css/bootstrap-theme.css")); ?>">
<link rel="stylesheet" href="<?php echo e(url("/assets/css/superfish.css")); ?>" media="screen">
<link rel="stylesheet" type="text/css" href="<?php echo e(url("/assets/css/slick.css")); ?>">
<link rel="stylesheet" type="text/css" href="<?php echo e(url("/assets/css/slick-theme.css")); ?>">
<link rel="stylesheet" type="text/css" href="<?php echo e(url('/assets/css/contact.css')); ?>">
<!-- SCRIPT -->
<script src="<?php echo e(url("/assets/js/jquery-3.0.0.min.js")); ?>"></script>
<script src="<?php echo e(url("/assets/js/jquery-migrate-3.0.0.min.js")); ?>"></script>
<script src="<?php echo e(url("/assets/js/superfish.js")); ?>"></script>
<script src="<?php echo e(url("/assets/js/slick.min.js")); ?>"></script>
<script src="<?php echo e(url("/assets/js/function.min.js")); ?>"></script>
<!-- Latest compiled and minified JavaScript -->
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js" integrity="<KEY>" crossorigin="anonymous"></script>
<?php echo $__env->yieldContent('head-scripts'); ?>
<?php if(Request::is('area/*')): ?>
<script src="http://maps.google.com/maps/api/js?sensor=false" type="text/javascript"></script>
<?php endif; ?>
</head><file_sep>/storage/framework/views/44a711a0e7520adeb4e40c8cde61ce5277a1ec39.php
<?php /**/ $title = $area[0]->title. ' Villa Rentals by Bali Home Paradise'; /**/ ?>
<?php $__env->startSection('title', $title); ?>
<?php $__env->startSection('content'); ?>
<div class="villa-category row" ng-app="frontend">
<?php if(isset($specialOffers)): ?>
<!-- SPECIAL OFFERS -->
<div id="special-offers" class="col-sm-4 col-xs-12">
<h1><a href="#special-offers">Special offers <span class="css-arrow-down"></span></a></h1>
<div class="arrow-down"><img src="<?php echo e(url("assets/images/icon-arrow-down.png")); ?>"></div>
<div id="special">
<?php foreach($specialOffers as $offer): ?>
<!-- villa-special -->
<div class="villa-special col-sm-12 col-xs-6">
<div class="img-villa"><img src="<?php echo e(URL::asset('assets/images/lazy-image.png')); ?>" lazy-img="<?php echo e(url('/thumb/'.$offer->original_name.'/350/temporary')); ?>" alt=""></div>
<div class="villa-name"><h2><?php echo e($offer->villa_title); ?></h2></div>
<div class="about-villa">
<div class="row">
<div class="col-sm-12">
<?php echo e($offer->title); ?>
</div>
<div class="features col-sm-12">
<span> <i class="fa fa-bed" aria-hidden="true"></i> <?php echo e($offer->bedrooms_no > 1 ? $offer->bedrooms_no . ' Bedrooms' : $offer->bedrooms_no . ' Bedroom'); ?> </span>
<span> <i class="fa fa-users" aria-hidden="true"></i> <?php echo e($offer->occupied_max > 1 ? $offer->occupied_max . ' Peoples' : $offer->occupied_max . ' People'); ?> Max </span>
<span> <i class="fa fa-check-circle" aria-hidden="true"></i> <?php echo e($offer->environment_title); ?> </span>
</div>
<div class="col-sm-12">
<a class="btn btn-default" href="<?php echo e(url($offer->area_slug.'/'.$offer->slug.'.html')); ?>" role="button">VIEW DETAIL</a>
</div>
</div>
</div>
</div>
<?php endforeach; ?>
</div>
</div>
<?php else: ?>
<div id="special-offers" class="col-sm-4 col-xs-12">
<h1>No Special Offer available yet</h1>
</div>
<?php endif; ?>
<!-- /SPECIAL OFFERS -->
<!-- CATEGORY -->
<div id="detail-category" class="col-sm-8 col-xs-12" ng-controller="homeController">
<h1> <?php echo e($area[0]->title); ?> </h1>
<?php if(!empty($area[0]->description)): ?>
<p><?php echo e($area[0]->description); ?></p>
<?php endif; ?>
<!-- villa-category -->
<div class="villa-category row" ng-init="getVillas(<?php echo e($area[0]->id); ?>)">
<!-- villa-item -->
<div infinite-scroll="getMoreData()">
<div class="villa-item col-sm-6" ng-repeat="villa in data">
<div class="img-villa"><img src="<?php echo e(URL::asset('assets/images/lazy-image.png')); ?>" lazy-img="{{ villa.thumbnail }}" alt=""></div>
<div class="rate">
<span> From </span>
<div class="price"> $ {{ villa.rate }} <span>/night</span></div>
</div>
<div class="about-villa">
<div class="villa-name"><h2>{{ villa.title }}</h2></div>
<div class="short-description">{{ villa.desc | limitTo: 100 | stripTags}}
</div>
<div class="features row">
<div class="features-1 col-sm-6">
<span> <i class="fa fa-bed" aria-hidden="true"></i> {{ villa.bedroom }} Bedroom </span>
<span> <i class="fa fa-users" aria-hidden="true"></i> {{ villa.occupied }} Max </span>
</div>
<div class="features-2 col-sm-6">
<span> <i class="fa fa-check-circle" aria-hidden="true"></i> {{ villa.environment }} </span>
<span> <i class="fa fa-star" aria-hidden="true"></i> {{ villa.review }} Review </span>
</div>
</div>
</div>
<a class="btn btn-default" href="{{ villa.url }}" role="button">VIEW DETAIL</a>
</div>
</div>
<!-- villa-item -->
</div>
<!-- /villa-category -->
</div>
<!-- /DETAIL CATEGORY -->
</div>
<?php $__env->stopSection(); ?>
<?php $__env->startSection('mapjs'); ?>
<script type="text/javascript" src="https://maps.googleapis.com/maps/api/js?key=<KEY>&libraries=places"></script>
<script type="text/javascript">
var locations = [
<?php foreach($villas as $villa): ?>
['<?php echo e($villa->title); ?>', <?php echo e($villa->latitude); ?>, <?php echo e($villa->longitude); ?>],
<?php endforeach; ?>
];
var map = new google.maps.Map(document.getElementById('map'), {
zoom: 14,
center: new google.maps.LatLng(<?php echo e($area[0]->latitude); ?>, <?php echo e($area[0]->longitude); ?>),
mapTypeId: google.maps.MapTypeId.ROADMAP
});
var infowindow = new google.maps.InfoWindow();
var marker, i;
for (i = 0; i < locations.length; i++) {
marker = new google.maps.Marker({
position: new google.maps.LatLng(locations[i][1], locations[i][2]),
map: map
});
google.maps.event.addListener(marker, 'click', (function(marker, i) {
return function() {
infowindow.setContent(locations[i][0]);
infowindow.open(map, marker);
}
})(marker, i));
}
</script>
<?php $__env->stopSection(); ?>
<?php echo $__env->make('partial.layout-category', array_except(get_defined_vars(), array('__data', '__path')))->render(); ?><file_sep>/storage/framework/views/d15899e93873cf39a9f07603743edf69042914b9.php
<?php $__env->startSection('title', 'Contact Us'); ?>
<?php $__env->startSection('head-scripts'); ?>
<?php $__env->stopSection(); ?>
<?php $__env->startSection('content'); ?>
<div class="container">
<div class="jumbotron">
<h1>Thanks a lot!</h1>
<h2>Please check your email for contract.</h2>
</div>
</div>
<?php $__env->stopSection(); ?>
<?php echo $__env->make('partial.layout-contact', array_except(get_defined_vars(), array('__data', '__path')))->render(); ?><file_sep>/storage/framework/views/ac89b9468779b2bbec9f8a5c51d60cd192e2ac33.php
<!-- CONTAINER-FLUID -->
<?php
use App\Area;
use App\Bedroom;
?>
<div class="container-fluid">
<!-- HEADER -->
<div id="header">
<!-- TOP-BAR -->
<div id="top-bar" class="row">
<div class="navigation col-sm-9">
<a href="##" class="toggle-nav"></a>
<?php echo $__env->make('partial.menu', array_except(get_defined_vars(), array('__data', '__path')))->render(); ?>
</div>
</div>
<!-- /TOP-BAR -->
<!-- IMG-HEADER -->
<div id="img-header">
<img src="assets/images/img-header.jpg" alt="">
<img src="assets/images/img-header-2.jpg" alt="">
<img src="assets/images/img-header-3.jpg" alt="">
</div>
<!-- /IMG-HEADER -->
<!-- VILLA-SEARCH -->
<div id="villa-search" class="clearfix">
<?php echo Form::open(['action'=>'SearchController@Index', 'method'=>'get']); ?>
<?php echo Form::token(); ?>
<div class="form-group col-md-3 col-xs-12 col-md-offset-1">
<input type="text" class="form-control" name="villa-name" id="exampleInputName2" placeholder="Villa Name">
</div>
<div class="col-md-3 col-xs-5">
<?php echo e(Form::select('bedroom-id', Bedroom::orderby('title')->lists('title','id')->prepend('Please select Bedroom'),null, ['class' => 'form-control'])); ?>
</div>
<div class="col-md-3 col-xs-5">
<?php echo e(Form::select('area-id', Area::lists('title','id')->prepend('Please selct Area'), null, ['class' => 'form-control'])); ?>
</div>
<div class=" col-xs-2 col-md-1">
<button type="submit" class="btn btn-default">Search</button>
</div>
<?php echo Form::close(); ?>
</div>
<!-- /VILLA-SEARCH -->
</div>
<!-- /HEADER -->
</div>
<!-- /CONTAINER-FLUID --><file_sep>/storage/framework/views/bca6da6daf71ab76859dcc2aa68fa3b3abc981dc.php
<!-- CONTAINER-FLUID -->
<div ng-app="frontend">
<div class="container-fluid" ng-controller="homeController">
<!-- HEADER -->
<div id="header">
<!-- TOP-BAR -->
<?php echo $__env->make('partial.menu', array_except(get_defined_vars(), array('__data', '__path')))->render(); ?>
<!-- /TOP-BAR -->
<!-- IMG-HEADER -->
<div id="header-villa">
<img src="<?php echo e(URL::asset('assets/images/lazy-image-large.png')); ?>" alt="<?php echo e(isset($villa) ? $villa[0]->title : ''); ?>" lazy-img="<?php echo e(!empty($ImageDetail) ?
url('/thumb-detail/'.$ImageDetail[0]->original_name.'/1280') : ''); ?>">
</div>
<!-- /IMG-HEADER -->
</div>
<!-- /HEADER -->
</div>
<!-- /CONTAINER-FLUID --><file_sep>/storage/framework/views/5317ff8c2b9e94587d7c553d07d997fd68d5962f.php
<nav class="navbar navbar-default navbar-fixed-top">
<div class="container">
<div class="navbar-header">
<button type="button" class="navbar-toggle collapsed" data-toggle="collapse" data-target="#navbar" aria-expanded="false" aria-controls="navbar">
<span class="sr-only">Toggle navigation</span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
</button>
<a href="<?php echo e(url('/temporary')); ?>" class="navbar-brand"> <img src="<?php echo e(asset('assets/images/new_logo.png')); ?>" style="width:60px; height:30px;"/> </a>
</div>
<div id="navbar" class="navbar-collapse collapse">
<ul class="nav navbar-nav navbar-right">
<li><a href="<?php echo e(url('/temporary')); ?>"> Home </a></li>
<li class="dropdown">
<a href="#" class="dropdown-toggle" data-toggle="dropdown" role="button" aria-haspopup="true" aria-expanded="false"> Villa By Area <span class="caret"></span></a>
<ul class="dropdown-menu">
<?php foreach($areas as $area): ?>
<li><a href="<?php echo e(url('/area/'. $area->slug)); ?>"> <?php echo e($area->title); ?> </a></li>
<?php endforeach; ?>
</ul>
</li>
<li><a href="<?php echo e(url('/contact-us')); ?>"> Contact Us </a></li>
</ul>
</div><!--/.nav-collapse -->
</div>
</nav><file_sep>/storage/framework/views/9aaafaf6d65f456af66475163636d1e5af82d690.php
<?php $__env->startSection('title', 'Bali Home Paradise'); ?>
<?php $__env->startSection('content'); ?>
<!-- WELCOME -->
<div ng-app="frontend">
<div id="welcome" class="container-fluid">
<div class="description">
<h1> Bali Home Paradise - Luxury Villas For Rent </h1>
Bali Home Paradise has been here helping many discerning guests to find their perfect Bali villas for almost a decade. While assisting, we also keep updating villa listings to give you, our valued customer, better options to choose. Today, we have been compiling a select amount of luxury and private villas for rent in many destinations in Bali. With a simple click you can select one of the best villas in Seminyak, Ubud, Canggu, and other popular destinations.
</div>
</div>
<!-- /WELCOME -->
<!-- FEATURED-VILLAS -->
<div id="featured-villas" ng-controller="homeController">
<div class="container">
<div class="row">
<h1> Featured Villas </h1>
<!-- villa-item -->
<?php foreach( $featuredVillas as $featuredVilla): ?>
<div class="villa-item col-sm-4">
<?php
if ($featuredVilla->gallery->isEmpty()) {
$image_url = 'assets/images/lazy-image.png';
} else {
foreach ($featuredVilla->gallery as $gallery) {
$image_url = '/thumb/'. $gallery->original_name .'/350/home';
}
}
?>
<div class="img-villa"><a href="<?php echo e(url($featuredVilla->area->slug.'/'.$featuredVilla->slug.'.html')); ?>"><img src="<?php echo e(URL::asset('assets/images/lazy-image.png')); ?>" lazy-img="<?php echo e(url($image_url)); ?>" alt=""></a></div>
<div class="rate">
<span> From </span>
<div class="price">
<?php echo e('$ '.(int)($featuredVilla->min_rate())); ?>
<span>/night</span></div>
</div>
<div class="about-villa">
<div class="villa-name"><h2><?php echo e($featuredVilla->title); ?></h2></div>
<div class="short-description"><?php echo e(str_limit(strip_tags($featuredVilla->intro), 125)); ?>
</div>
<div class="features row">
<div class="features-1 col-sm-6">
<span> <i class="fa fa-bed" aria-hidden="true"></i> <?php echo e($featuredVilla->bedrooms_no > 1 ? $featuredVilla->bedrooms_no . ' Bedrooms' : $featuredVilla->bedrooms_no . ' Bedroom'); ?></span>
<span> <i class="fa fa-users" aria-hidden="true"></i> <?php echo e($featuredVilla->occupied_max > 1 ? $featuredVilla->occupied_max . ' Peoples' : $featuredVilla->occupied_max . ' People'); ?> </span>
</div>
<div class="features-2 col-sm-6">
<span> <i class="fa fa-check-circle" aria-hidden="true"></i> <?php echo e($featuredVilla->environment->title); ?> </span>
<span> <i class="fa fa-star" aria-hidden="true"></i> <?php echo e(count($featuredVilla->review) > 1 ? count($featuredVilla->review) . ' Reviews' : count($featuredVilla->review) . ' Review'); ?> </span>
</div>
</div>
</div>
<a class="btn btn-default" href="<?php echo e(url($featuredVilla->area->slug.'/'.$featuredVilla->slug.'.html')); ?>" style="background-color:#e0e0e0!important;">VIEW DETAIL</a>
</div>
<?php endforeach; ?>
</div>
</div>
</div>
<!-- /FEATURED-VILLAS -->
<!-- TESTIMONIAL -->
<div id="testimonial-guest">
<div class="container">
<h1> Guest Testimonial </h1>
<div class="testimonial row">
<?php foreach($testimonials as $testimonial): ?>
<div class="guest-testi col-sm-4">
<h2> <?php echo e($testimonial->guest_name); ?> </h2>
<span> <?php echo e(!empty($testimonial->city) ? $testimonial->city .' - ' : ''); ?> <?php echo e($testimonial->country->name); ?> </span>
<q><?php echo e(str_limit(strip_tags($testimonial->comments), 122)); ?></q>
</div>
<?php endforeach; ?>
</div>
</div>
</div>
<!-- /TESTIMONIAL -->
</div>
<?php $__env->stopSection(); ?>
<?php echo $__env->make('partial.layout', array_except(get_defined_vars(), array('__data', '__path')))->render(); ?><file_sep>/storage/framework/views/2f7c1a39b4b557862819a3be99c2b95b811bd84b.php
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1, user-scalable=no">
<title><?php echo $__env->yieldContent('title'); ?></title>
<!-- FONT -->
<link href='https://fonts.googleapis.com/css?family=Biryani:400,200,300,700' rel='stylesheet' type='text/css'>
<link href='https://fonts.googleapis.com/css?family=Lato:400,100,300,700' rel='stylesheet' type='text/css'>
<link rel="stylesheet" href="<?php echo e(URL::asset('assets/css/font-awesome.min.css')); ?>">
<!-- CSS -->
<link rel="stylesheet" type="text/css" href="<?php echo e(URL::asset('assets/css/style.css')); ?>">
<link rel="stylesheet" href="<?php echo e(url("/assets/css/bootstrap-theme.css")); ?>">
<link rel="stylesheet" href="<?php echo e(URL::asset('assets/css/superfish.css')); ?>" media="screen">
<link rel="stylesheet" type="text/css" href="<?php echo e(URL::asset('assets/css/slick.css')); ?>">
<link rel="stylesheet" type="text/css" href="<?php echo e(URL::asset('assets/css/slick-theme.css')); ?>">
<link rel="stylesheet" type="text/css" href="<?php echo e(URL::asset('assets/css/frontend.css')); ?>">
<link rel="stylesheet" type="text/css" href="<?php echo e(URL::asset('assets/css/datepicker.css')); ?>">
<!-- SCRIPT -->
<script src="<?php echo e(URL::asset('assets/js/jquery-3.0.0.min.js')); ?>"></script>
<script src="<?php echo e(URL::asset('assets/js/jquery-migrate-3.0.0.min.js')); ?>"></script>
<script src="<?php echo e(URL::asset('assets/js/superfish.js')); ?>"></script>
<script src="<?php echo e(URL::asset('assets/js/slick.min.js')); ?>"></script>
<!-- Latest compiled and minified JavaScript -->
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js" integrity="<KEY>" crossorigin="anonymous"></script>
<script src="<?php echo e(URL::asset('assets/js/bootstrap-datepicker.js')); ?>"></script>
<script src="<?php echo e(URL::asset('assets/js/function.min.js')); ?>"></script>
<script src="http://maps.google.com/maps/api/js?sensor=false" type="text/javascript"></script>
<!-- <script src='https://www.google.com/recaptcha/api.js'></script> -->
<script type="text/javascript" src="https://maps.googleapis.com/maps/api/js?key=<KEY>&libraries=places"></script>
<style>
.g-recaptcha {
transform-origin: left top;
-webkit-transform-origin: left top;
}
.captcha-container {
max-width: 300px;
padding: 20px;
}
.footer {
position: relative;
bottom: 0;
width: 100%;
/* Set the fixed height of the footer here */
height: 100px;
background-color: #f5f5f5;
}
</style>
</head><file_sep>/storage/framework/views/a506ec9a1005c0dc5250d17bae31b827bf0b0d6f.php
<?php /**/ $title = $villa[0]->title. ' | '.$area[0]->title. ' villa rentals'; /**/ ?>
<?php $__env->startSection('title', $title); ?>
<?php $__env->startSection('content'); ?>
<!-- villa-detail -->
<div class="villa-detail col-sm-12" ng-app="frontend">
<!-- QUICK CONTACT -->
<div class="modal fade" id="myModal" tabindex="-1" role="dialog" aria-labelledby="myModalLabel">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-header">QUICK CONTACT</div>
<div class="modal-body">
<div class="col-xs-12">
<form class="form-horizontal" action="<?php echo e(url('detail/order')); ?>" method="post">
<?php echo Form::token(); ?>
<input type="hidden" name="villa-id" value="<?php echo e($villa[0]->id); ?>"/>
<div id="form-contact-wrapper">
<div class="form-group">
<div class="row">
<label style="width:80px;">Checkin: </label>
<a href="#" class="btn small dp4" id="dp4" data-date-format="yyyy-mm-dd" data-date="2017-02-01"><i class="fa fa-calendar"></i></a>
<input name="check-in" id="startDate" value="2017-02-01"/>
</div>
<div class="row">
<label style="width:80px;">Checkout: </label>
<a href="#" class="btn small dp5" id="dp5" data-date-format="yyyy-mm-dd" data-date="2017-02-10"><i class="fa fa-calendar"></i></a>
<input name="check-out" id="endDate" value="2017-02-10"/>
</div>
</div>
<div class="form-group number-guest">
<label>Number Of Guest </label>
<div class="row">
<input type="number" min="1" class="form-control col-sm-6" name="num-guest" placeholder="Adult">
<input type="number" min="1" class="form-control col-sm-6" name="num-child" placeholder="Child">
</div>
</div>
<div class="form-group">
<input class="form-control" name="user-name" placeholder="Name">
</div>
<div class="form-group">
<input type="email" class="form-control" name="user-email" id="exampleInputEmail1" placeholder="Email">
</div>
<div class="form-group">
<input class="form-control" name="user-phone" placeholder="Phone">
</div>
<button type="submit" class="btn btn-default btn-submit" style="margin:0px 10%; width:80%;">Order Now</button>
</div>
</form>
</div>
<div class="clearfix"></div>
</div>
</div>
</div>
</div>
<div id="quick-contact" class="col-sm-3 contact-box hidden-sm hidden-xs">
<form class="form-horizontal" action="<?php echo e(url('detail/order')); ?>" method="post">
<?php echo Form::token(); ?>
<input type="hidden" name="villa-id" value="<?php echo e($villa[0]->id); ?>"/>
<h1><a href="#quick-contact">QUICK CONTACT <span class="css-arrow-down"></span></a></h1>
<div class="arrow-down"><img src="<?php echo e(URL::asset('assets/images/icon-arrow-down.png')); ?>"></div>
<div id="form-contact-wrapper">
<div class="form-group">
<div class="row">
<label style="width:80px;">Checkin: </label>
<a href="#" class="btn small dp4" id="dp4" data-date-format="yyyy-mm-dd" data-date="2017-02-01"><i class="fa fa-calendar"></i></a>
<input name="check-in" id="startDate" value="2017-02-01"/>
</div>
<div class="row">
<label style="width:80px;">Checkout: </label>
<a href="#" class="btn small dp5" id="dp5" data-date-format="yyyy-mm-dd" data-date="2017-02-10"><i class="fa fa-calendar"></i></a>
<input name="check-out" id="endDate" value="2017-02-10"/>
</div>
</div>
<div class="form-group number-guest">
<label>Number Of Guest </label>
<div class="row">
<input type="number" min="1" class="form-control col-sm-6" name="num-guest" placeholder="Adult">
<input type="number" min="1" class="form-control col-sm-6" name="num-child" placeholder="Child">
</div>
</div>
<div class="form-group">
<input class="form-control" name="user-name" placeholder="Name">
</div>
<div class="form-group">
<input type="email" class="form-control" name="user-email" id="exampleInputEmail1" placeholder="Email">
</div>
<div class="form-group">
<input class="form-control" name="user-phone" placeholder="Phone">
</div>
<div class="form-group verification-code captcha-container" style="margin:0 auto !important;">
<div class="g-recaptcha" data-sitekey="<?php echo e(env('RE_CAP_SITE')); ?>" data-callback="capenable" data-expired-callback="capdisable"></div>
</div>
<button type="submit" class="btn btn-default btn-submit" style="margin:0px 10%; width:80%;">Order Now</button>
</div>
</form>
</div>
<!-- /QUICK CONTACT -->
<!-- DETAIL VILLA -->
<div id="detail-villa" class="col-sm-9" ng-controller="homeController">
<h1> <?php echo e(strtoupper($villa[0]->title)); ?> </h1>
<?php if(isset($villa[0]->intro)): ?>
<?php echo $villa[0]->intro; ?> <a href="#">More Detail</a>
<?php else: ?>
<?php echo str_limit($villa[0]->description, 300); ?> <a href="#">More Detail</a>
<?php endif; ?>
<!-- Tabs -->
<div id="villa-tabs">
<div id="villa-tabs-nav" class="btn-group text-center" role="group" aria-label="...">
<a href="#location" class="btn btn-default" role="button">Location</a>
<a href="#gallery" class="btn btn-default active" role="button">Gallery</a>
<a href="#rates" class="btn btn-default" role="button">Rates</a>
<a href="#services" class="btn btn-default" role="button">Services & Facilities</a>
<a href="#staff" class="btn btn-default" role="button">Staff Detail</a>
<a href="#reviews" class="btn btn-default" role="button">Reviews</a>
</div>
<div class="resp-tabs-container hor_1">
<!-- location -->
<div id="location" class="section">
<h2>Location</h2>
<div id="villa-map" style="width: 100%; height: 300px"></div>
</div>
<!-- gallery -->
<div>
<div id="gallery" class="section" ng-init="getGalleryByVilla(<?= $villa[0]->id ?>)">
<h2> Gallery </h2>
<div class="gallery-image">
<span ng-repeat="gallery in galleryByVilla">
<a href="{{ gallery.url }}" data-lightbox="{{ gallery.name }}" data-title="{{ gallery.caption }}"><img src="<?php echo e(URL::asset('assets/images/lazy-image-detail.png')); ?>" lazy-img="{{ gallery.thumbUrl }}" /></a>
</span>
</div>
</div>
</div>
<!-- rates -->
<div id="rates" class="section">
<h2>Rates</h2>
<div class="rates-detail">
<?php if(isset($rates)): ?>
<div class="table-responsive">
<?php foreach($rates as $key => $value): ?>
<table class="table">
<thead>
<th width="50%"></th>
<th>Minimum Stay</th>
<th><?php echo e($key); ?></th>
</thead>
<?php foreach($value as $rate): ?>
<tr>
<td width="50%"> <?php echo e($rate->season); ?></td>
<td><?php echo e(isset($rate->min_stay) ? $rate->min_stay . ' Nights' : ''); ?></td>
<td>USD <?php echo e($rate->rate); ?> <?php echo e($rate->plus === 1 ? '++' : ''); ?> <?php echo e(isset($rate->tax) ? 'Tax: '.str_replace('.00', '%', $rate->tax) : ''); ?></td>
</tr>
<?php endforeach; ?>
</table>
<?php endforeach; ?>
</div>
<?php endif; ?>
</div>
</div>
<!-- services & facilites -->
<div id="services" class="section">
<h2>Services & Facilities</h2>
<div class="services-detail">
<?php if(!empty($villa[0]->services) || !empty($villa[0]->facilities)): ?>
<?php echo $villa[0]->services; ?>
<?php echo $villa[0]->facilities; ?>
<?php endif; ?>
</div>
</div>
<div id="staff" class="section">
<h2>Staff Detail</h2>
<div class="services-detail">
<?php if(!empty($villa[0]->staff_detail)): ?>
<?php echo $villa[0]->staff_detail; ?>
<?php endif; ?>
</div>
</div>
<!-- reviews -->
<div id="reviews" class="section" ng-init="getReviewByVilla(<?= $villa[0]->id ?>)">
<h2>Reviews</h2>
<div class="reviews-detail" ng-hide="reviewStatus">
<div class="panel panel-default" ng-repeat="review in reviewByVilla">
<div class="panel-heading">{{ review.guestName }}, from {{ review.guestCountry }}</div>
<div class="panel-body">
{{ review.guestComment | stripTags }}
</div>
</div>
</div>
<div class="reviews-detail" ng-show="reviewStatus">
<p class="text-center">No reviews available</p>
</div>
</div>
</div>
</div>
<!-- /Tabs -->
</div>
<!-- /DETAIL VILLA -->
</div>
<!-- /villa-detail -->
<?php $__env->stopSection(); ?>
<?php $__env->startSection('mapjs'); ?>
<script src="<?php echo e(URL::asset('assets/js/jquery.throttle.js')); ?>"></script>
<script type="text/javascript">
var scaleCaptcha = function(elementWidth) {
// Width of the reCAPTCHA element, in pixels
var reCaptchaWidth = 304;
// Get the containing element's width
var containerWidth = $('.captcha-container').width();
// Only scale the reCAPTCHA if it won't fit
// inside the container
if(reCaptchaWidth > containerWidth) {
// Calculate the scale
var captchaScale = containerWidth / reCaptchaWidth;
// Apply the transformation
$('.g-recaptcha').css({
'transform':'scale('+captchaScale+')'
});
}
};
function capenable() {
$('.btn-submit').prop('disabled', false);
}
function capdisable() {
$('.btn-submit').prop('disabled', true);
}
var fixmeTop = $('.contact-box').offset().top; // get initial position of the element
$(window).scroll(function() { // assign scroll event listener
var currentScroll = $(window).scrollTop(); // get current position
if (currentScroll >= fixmeTop) { // apply position: fixed if you
$('.contact-box').css({ // scroll to that element or below it
position: 'fixed',
top: '50px',
left: '15px'
});
}
else { // apply position: static
$('.contact-box').css({ // if you scroll above it
position: 'fixed',
top: $("#header-villa").height()+50-currentScroll,
left: '15px'
});
}
$("#detail-villa").addClass('col-sm-offset-3');
});
var locations = [
['<?php echo e($villa[0]->title); ?>', <?php echo e($villa[0]->latitude); ?>, <?php echo e($villa[0]->longitude); ?>],
];
var map = new google.maps.Map(document.getElementById('villa-map'), {
zoom: 14,
center: new google.maps.LatLng(<?php echo e($area[0]->latitude); ?>, <?php echo e($area[0]->longitude); ?>),
mapTypeId: google.maps.MapTypeId.ROADMAP
});
var infowindow = new google.maps.InfoWindow();
var marker, i;
for (i = 0; i < locations.length; i++) {
marker = new google.maps.Marker({
position: new google.maps.LatLng(locations[i][1], locations[i][2]),
map: map
});
google.maps.event.addListener(marker, 'click', (function(marker, i) {
return function() {
infowindow.setContent(locations[i][0]);
infowindow.open(map, marker);
}
})(marker, i));
}
</script>
<script>
$(function(){
$('#body').removeClass('container');
//capdisable();
scaleCaptcha();
$(window).resize( $.throttle( 100, scaleCaptcha ) );
$('.dp4').datepicker()
.on('changeDate', function(ev){
if (ev.date.valueOf() > endDate.valueOf()){
$('#alert').show().find('strong').text('The start date can not be greater then the end date');
} else {
$('#alert').hide();
startDate = new Date(ev.date);
$('#startDate').val($('#dp4').data('date'));
}
$('#dp4').datepicker('hide');
});
$('.dp5').datepicker()
.on('changeDate', function(ev){
if (ev.date.valueOf() < startDate.valueOf()){
$('#alert').show().find('strong').text('The end date can not be less then the start date');
} else {
$('#alert').hide();
endDate = new Date(ev.date);
$('#endDate').val($('#dp5').data('date'));
}
$('#dp5').datepicker('hide');
});
});
</script>
<?php $__env->stopSection(); ?>
<?php echo $__env->make('partial.layout-detail', array_except(get_defined_vars(), array('__data', '__path')))->render(); ?><file_sep>/storage/framework/views/354454e63f3ebb55c8b650f7d6ee866eecf9bffb.php
<?php $__env->startSection('title', 'Contact Us'); ?>
<?php $__env->startSection('head-scripts'); ?>
<script src="http://maps.google.com/maps/api/js?sensor=false" type="text/javascript"></script>
<?php $__env->stopSection(); ?>
<?php $__env->startSection('content'); ?>
<div class="row">
<div class="col-md-9 col-sm-8">
<div class="contact-bali-symbol">
<i class="fa fa-bank"></i>
</div>
<div class="help row">
<div class="col-sm-12">
<h2>About Us</h2>
<p>
In July 2015 UKIN Business Consulting based in Jakarta, Indonesia, with a branch office in Denpasar, Bali, acquired and now manages Bali Home Paradise, which has been established in 2008. UKIN Consulting specializes in tourism related services with a focus on business analysis, hospitality management and training.
UKIN is committed to provide the guest and clients of Bali Home Paradise comprehensive and international-level hospitality service along the complete journey. We are highly dedicated to make every holiday a unique and lasting experience. Our commitment goes beyond your vacation at one of our villas. We will gladly welcome back and strive to make your stay even more pleasant and memorable. We also offer a reward program for loyal customers.
</p><br/><br/><br/><br/>
<h2>Bali Home Paradise</h2>
<p> Managed By.
PT. Unik Kreatif Inovatif (UKIN)
World Trade Centre 5 Level 3A
Jl. Jendral Sudirman Kav. 29 - 31
Jakarta 12920
Indonesia<br/>
<strong>Telp. +62 21 2598 5188</strong>
<br/>
<strong>Fax. +62 21 2598 5001</strong>
</p>
</div>
</div>
</div>
<div class="col-md-3 col-sm-4">
<div id="villa-map" style="width: 100%; height: 300px"></div>
<br/>
<img src
<p class="text-center">Managed By: <br/>
PT. Unik Kreatif Inovatif</p>
<p class="text-center">Jl. <NAME> O No. 17 <br/>
Denpasar 80226 <br/>
Bali</p>
</div>
</div>
<?php $__env->stopSection(); ?>
<?php $__env->startSection('scripts'); ?>
<script type="text/javascript">
var locations = [
['PT. Unik Kreatif Inovatif', -6.2116791, 106.8209164],
];
var map = new google.maps.Map(document.getElementById('villa-map'), {
zoom: 14,
center: new google.maps.LatLng(-6.2116791, 106.8209164),
mapTypeId: google.maps.MapTypeId.ROADMAP
});
var infowindow = new google.maps.InfoWindow();
var marker, i;
for (i = 0; i < locations.length; i++) {
marker = new google.maps.Marker({
position: new google.maps.LatLng(-6.2116791,106.8209164),
map: map
});
google.maps.event.addListener(marker, 'click', (function(marker, i) {
return function() {
infowindow.setContent(locations[i][0]);
infowindow.open(map, marker);
}
})(marker, i));
}
</script>
<script src="<?php echo e(asset("/assets/js/vendor-frontend.js")); ?>"></script>
<script src="<?php echo e(asset("/assets/js/frontend/app.js")); ?>"></script>
<?php $__env->stopSection(); ?>
<?php echo $__env->make('partial.layout-contact', array_except(get_defined_vars(), array('__data', '__path')))->render(); ?><file_sep>/storage/framework/views/fb92e128976d876712bd18e77f76db40e710fd97.php
<!-- Header -->
<?php echo $__env->make('partial/header-detail', array_except(get_defined_vars(), array('__data', '__path')))->render(); ?>
<body>
<div style="position:fixed; top:50px; left:0; width:100%; z-index:999;" class="hidden-md hidden-lg">
<button type="button" class="btn btn-primary btn-lg col-xs-12" data-toggle="modal" data-target="#myModal">
QUICK CONTACT
</button>
</div>
<div style="height:85px;" class="hidden-md hidden-lg"></div>
<?php echo $__env->make('partial/nav-detail', array_except(get_defined_vars(), array('__data', '__path')))->render(); ?>
<!-- BODY -->
<div id="body" class="container">
<?php echo $__env->yieldContent('content'); ?>
</div>
</div>
<!-- /BODY -->
<!-- CONTAINER-FLUID -->
<?php echo $__env->make('partial/footer', array_except(get_defined_vars(), array('__data', '__path')))->render(); ?>
<?php echo $__env->yieldContent('mapjs'); ?>
<!-- CONTAINER-FLUID -->
<script src="<?php echo e(asset("/assets/js/vendor-frontend.js")); ?>"></script>
<script src="<?php echo e(asset("/assets/js/frontend/app.js")); ?>"></script>
</body>
</html><file_sep>/storage/framework/views/6c369416b77f76d2645deeba3ca2f0b03ef4d375.php
<!-- CONTAINER-FLUID -->
<div class="container-fluid">
<!-- HEADER -->
<div id="header">
<!-- TOP-BAR -->
<div id="top-bar" class="row">
<div class="navigation col-sm-9">
<a href="##" class="toggle-nav"></a>
<?php echo $__env->make('partial.menu', array_except(get_defined_vars(), array('__data', '__path')))->render(); ?>
</div>
</div>
<!-- /TOP-BAR -->
<!-- IMG-HEADER -->
<div id="map" style="width: 100%; height: 400px;">
</div>
<!-- /IMG-HEADER -->
<!-- SEARCH-RESULT -->
<div id="search-result">
Category: <span><?php echo e($area[0]->title); ?> Villas</span>
</div>
<!-- /SEARCH-RESULT -->
</div>
<!-- /HEADER -->
</div>
<!-- /CONTAINER-FLUID --><file_sep>/storage/framework/views/fd8d13011ad8fe79f43ae4b290c70cbe0bc028e7.php
<!-- Header -->
<?php echo $__env->make('partial/header', array_except(get_defined_vars(), array('__data', '__path')))->render(); ?>
<body>
<?php echo $__env->make('partial/nav-category', array_except(get_defined_vars(), array('__data', '__path')))->render(); ?>
<!-- BODY -->
<div id="body" class="container">
<?php echo $__env->yieldContent('content'); ?>
</div>
<!-- /BODY -->
<!-- CONTAINER-FLUID -->
<?php echo $__env->make('partial/footer', array_except(get_defined_vars(), array('__data', '__path')))->render(); ?>
<!-- CONTAINER-FLUID -->
<?php echo $__env->yieldContent('mapjs'); ?>
<script src="<?php echo e(asset("/assets/js/vendor-frontend.js")); ?>"></script>
<script src="<?php echo e(asset("/assets/js/frontend/app.js")); ?>"></script>
</body>
</html><file_sep>/storage/framework/views/0b6a18a00c8cbd748767994c1cebd00f89fba7be.php
<?php
use App\Area;
use App\Bedroom;
?>
<?php $__env->startSection('title', 'Search'); ?>
<?php $__env->startSection('content'); ?>
<div class="container">
<div id="villa-search" class="clearfix">
<?php echo Form::open(['action'=>'SearchController@Index', 'method'=>'get', 'id'=>'form-search']); ?>
<?php echo Form::token(); ?>
<div class="form-group col-sm-3 col-xs-12">
<input type="text" class="form-control" name="villa-name" id="exampleInputName2" placeholder="Villa Name" value="<?php echo e($vname); ?>">
</div>
<input type="hidden" name="sort-type" id="sort-type" value="<?php echo e($sort_type); ?>"/>
<div class="col-sm-3 col-xs-6">
<?php echo e(Form::select('bedroom-id', Bedroom::lists('title','id')->prepend('Bedroom'), $bedroom_id, ['class' => 'form-control'])); ?>
</div>
<div class="col-sm-3 col-xs-6">
<?php echo e(Form::select('area-id', Area::lists('title','id')->prepend('Area'), $area_id, ['class' => 'form-control'])); ?>
</div>
<div class="col-sm-1 col-xs-6">
<button type="submit" class="btn btn-default">Search</button>
</div>
<div class="btn-group group-sort col-md-2 col-xs-6" role="group" aria-label="...">
<button type="button" class="btn btn-default sort-no"><i class="fa fa-close"></i></button>
<button type="button" class="btn btn-default sort-asc"><i class="fa fa-sort-amount-asc" aria-hidden="true"></i></button>
<button type="button" class="btn btn-default sort-desc"><i class="fa fa-sort-amount-desc" aria-hidden="true"></i></button>
</div>
<?php echo Form::close(); ?>
</div>
<!-- /VILLA-SEARCH -->
<div ng-app="frontend">
<div class="row">
<hr/>
<!-- villa-item -->
<?php foreach( $villas as $villa): ?>
<div class="villa-item col-sm-12 col-md-4">
<?php
if ($villa->gallery->isEmpty()) {
$image_url = 'assets/images/lazy-image.png';
} else {
foreach ($villa->gallery as $gallery) {
$image_url = '/thumb/'. $gallery->original_name .'/350/home';
}
}
?>
<div class="img-villa"><a href="<?php echo e(url($villa->area->slug.'/'.$villa->slug.'.html')); ?>"><img src="<?php echo e(URL::asset('assets/images/lazy-image.png')); ?>" lazy-img="<?php echo e(url($image_url)); ?>" ></a></div>
<div class="rate">
<span> From </span>
<div class="price">
<?php echo e('$ '.(int)($villa->min_rate())); ?>
<span>/night</span></div>
</div>
<div class="about-villa">
<div class="villa-name"><h2><?php echo e($villa->title); ?></h2></div>
<div class="short-description"><?php echo e(str_limit(strip_tags($villa->intro), 125)); ?>
</div>
<div class="features row">
<div class="features-1 col-sm-6">
<span> <i class="fa fa-bed" aria-hidden="true"></i> <?php echo e($villa->bedrooms_no > 1 ? $villa->bedrooms_no . ' Bedrooms' : $villa->bedrooms_no . ' Bedroom'); ?></span>
<span> <i class="fa fa-users" aria-hidden="true"></i> <?php echo e($villa->occupied_max > 1 ? $villa->occupied_max . ' Peoples' : $villa->occupied_max . ' People'); ?> </span>
</div>
<div class="features-2 col-sm-6">
<span> <i class="fa fa-check-circle" aria-hidden="true"></i> <?php echo e($villa->environment->title); ?> </span>
<span> <i class="fa fa-star" aria-hidden="true"></i> <?php echo e(count($villa->review) > 1 ? count($villa->review) . ' Reviews' : count($villa->review) . ' Review'); ?> </span>
</div>
</div>
</div>
<a class="btn btn-default" href="<?php echo e(url($villa->area->slug.'/'.$villa->slug.'.html')); ?>" style="background-color:#e0e0e0!important;">VIEW DETAIL</a>
</div>
<?php endforeach; ?>
</div>
</div>
</div>
</div>
<?php $__env->stopSection(); ?>
<?php $__env->startSection("scripts"); ?>
<script>
$(function(){
var sort_type = $('#sort-type').val();
if (sort_type == "NO")
$('.sort-no').addClass('active');
if (sort_type == "ASC")
$('.sort-asc').addClass('active');
if (sort_type == "DESC")
$('.sort-desc').addClass('active');
});
$('.group-sort .sort-no').click(function(){
$('#sort-type').val('NO');
$('#form-search').submit();
});
$('.group-sort .sort-asc').click(function(){
$('#sort-type').val('ASC');
$('#form-search').submit();
});
$('.group-sort .sort-desc').click(function(){
$('#sort-type').val('DESC');
$('#form-search').submit();
});
</script>
<script src="<?php echo e(asset("/assets/js/vendor-frontend.js")); ?>"></script>
<script src="<?php echo e(asset("/assets/js/frontend/app.js")); ?>"></script>
<?php $__env->stopSection(); ?>
<?php echo $__env->make('partial.layout-contact', array_except(get_defined_vars(), array('__data', '__path')))->render(); ?><file_sep>/storage/framework/views/76fea5044679fe858af00f555cdf955ccafa1524.php
<!-- Header -->
<?php echo $__env->make('partial.header', array_except(get_defined_vars(), array('__data', '__path')))->render(); ?>
<body style="padding-top:70px;padding-bottom:30px;">
<?php echo $__env->make('partial/nav-contact', array_except(get_defined_vars(), array('__data', '__path')))->render(); ?>
<!-- BODY -->
<div id="body" class="container">
<?php echo $__env->yieldContent('content'); ?>
</div>
<!-- /BODY -->
<?php echo $__env->make('partial/footer', array_except(get_defined_vars(), array('__data', '__path')))->render(); ?>
<?php echo $__env->yieldContent("scripts"); ?>
</body>
</html><file_sep>/storage/framework/views/d7ccb2c8eff7b590b0c3f5f822bc1fa00485e8cf.php
<!DOCTYPE html>
<html lang="en-US">
<head>
<meta charset="utf-8">
</head>
<body>
<h2>Hi <?php echo e($user["name"]); ?></h2>
<a href="mailto:<?php echo e(env('EMAIL_SENDER')); ?>?Subject=Hello!&body=<?php echo e($content); ?>" target="_top">Send Mail</a>
<div>
Thanks for ordering.
In order to confirm your decision, Please send us your offer.
</div>
</body>
</html> | 9ff3317291b27df0ffa2a47d05c9487f11d2fb51 | [
"PHP"
] | 16 | PHP | nguyenthaison/bookinghotel | 789fe8cbba70ad8f001b6dc50ebd711f74c3e4f6 | eecf2f75f680b944fe74e25667a72e55ab46e826 | |
refs/heads/master | <file_sep>import torch
from torch.autograd import Variable
from parameterized import parameterized
from embzip.data import load_embeddings_txt, make_vocab
from embzip.model import gumbel_softmax, EmbeddingCompressor
from embzip.data import load_embeddings_txt, make_vocab, load_hdf5, save_hdf5
from embzip.model import (gumbel_softmax, EmbeddingCompressor,
FactorizedEmbeddingsOutput,
FactorizedEmbeddingsInput)
@parameterized([
('data/glove.6B.300d.txt', 100),
])
def test_glove_txt(path, maxsize):
gloves = load_embeddings_txt(path, maxsize)
assert len(gloves) <= maxsize
for k, v in gloves.items():
assert isinstance(k, str)
assert isinstance(v, torch.autograd.Variable)
assert v.data.shape[0] == 1
@parameterized([
('data/glove.6B.300d.txt', 100),
])
def test_vocab(path, maxsize):
gloves = load_embeddings_txt(path, maxsize)
vocab = make_vocab(gloves)
assert len(vocab['idx2word']) == \
len(vocab['word2idx']) == \
vocab['emb_tables'].data.shape[0]
@parameterized([
(None, 3, 4),
(2, 3, 4),
])
def test_one_hot(batch, n_dic, n_codes):
size = torch.Size([n_dic])
if batch is not None:
size = torch.Size([batch]) + size
t = torch.LongTensor(size).random_() % n_codes
o = one_hot(t, n_codes)
print(o)
exp_size = size + torch.Size([n_codes])
assert exp_size == o.size()
@parameterized([
(2, 3, 4)
])
def test_gumbel_softmax(b, m, k):
logits = Variable(1 * torch.Tensor(b * m, k).uniform_())
gsm = gumbel_softmax(logits, tau=1, hard=True)
print(gsm)
print(gsm.view(b, m * k))
assert torch.equal(torch.ones(b*m), torch.sum(gsm, -1).data)
@parameterized([
(2, 5, 2, 4)
])
def test_compressor(batch, emb_size, n_dic, n_codes):
ec = EmbeddingCompressor(emb_size, n_dic, n_codes)
inp = Variable(torch.Tensor(batch, emb_size).uniform_())
out = ec(inp)
print(out)
@parameterized([
('data/glove.6B.300d.txt', 100),
])
def test_save_hdf5(path, num_embs):
gloves, _ = load_embeddings_txt(path, num_embs)
ec = EmbeddingCompressor(300, 8, 16)
orig_embs, orig_vocab_map = save_hdf5('foo.h5', ec, gloves)
embs, vocab_map = load_hdf5('foo.h5')
assert(np.array_equal(orig_embs, embs))
assert orig_vocab_map == vocab_map
@parameterized([
([[0]], [[0, 1], [1, 2]]),
([[0, 1], [1, 2]], [[1, 2], [3, 2], [0, 3]]),
([[0, 1], [2, 1]], [[0, 1], [1, 2], [2, 3]])
])
def test_fe_input(indices, index_map):
vocab_map = {i: index_map[i] for i in range(len(index_map))}
emb_table = np.arange(10).reshape(5, 2)
indices = Variable(torch.LongTensor(indices))
print(indices)
fei = FactorizedEmbeddingsInput(emb_table, vocab_map)
out = fei(indices)
print(out)
# figure out expanded embeddings
index_map_t = torch.LongTensor(index_map)
index_map_t = torch.autograd.Variable(index_map_t)
exp_emb_table = torch.nn.Embedding(*emb_table.shape)
exp_emb_table.weight.data.copy_(torch.from_numpy(emb_table))
final_emb_table = exp_emb_table(index_map_t).sum(-2).data
et = torch.nn.Embedding(*final_emb_table.shape)
et.weight.data.copy_(final_emb_table)
exp_out = et(indices)
print(exp_out)
assert torch.equal(exp_out.data, out.data)
@parameterized([
([[1, 1]], [[0, 1], [1, 2]]),
([[0, 1], [1, 2]], [[1, 2], [3, 2], [0, 3]]),
([[0, 1]], [[0, 1], [1, 2], [2, 3]]),
([[0, 1], [1, 1]], [[0, 1], [1, 2], [2, 3]]),
([[0, 1], [1, 1], [1, 0]], [[0, 1], [1, 2], [2, 3]])
])
def test_fe_output(pe, index_map):
e = 2
mk = 5
v = len(index_map)
mk_table = np.arange(10).reshape(e, mk)
mk_table_T = np.arange(10).reshape(mk, e)
vocab_map = {i: index_map[i] for i in range(len(index_map))}
feo = FactorizedEmbeddingsOutput(np.array(mk_table), vocab_map)
print(mk_table_T)
emb_table = np.vstack([mk_table_T[index_map[i]].sum(0) for i in range(v)])
print('e', emb_table)
pe = Variable(torch.Tensor(pe))
out = feo(pe)
out_exp = pe.data @ torch.from_numpy(emb_table).float().t()
print('o', out_exp)
assert torch.equal(out.data, out_exp)
<file_sep>import torch
import torch.nn.functional as F
from torch.autograd import Variable
from torch import nn
def sample_gumbel(shape, eps=1e-10, out=None):
"""
Sample from Gumbel(0, 1)
based on
https://github.com/ericjang/gumbel-softmax/blob/3c8584924603869e90ca74ac20a6a03d99a91ef9/Categorical%20VAE.ipynb ,
(MIT license)
"""
U = out.resize_(shape).uniform_() if out is not None else torch.rand(shape)
return - torch.log(eps - torch.log(U + eps))
def gumbel_softmax_sample(logits, tau=1, eps=1e-10):
"""
Draw a sample from the Gumbel-Softmax distribution
based on
https://github.com/ericjang/gumbel-softmax/blob/3c8584924603869e90ca74ac20a6a03d99a91ef9/Categorical%20VAE.ipynb
(MIT license)
"""
gumbel_noise = sample_gumbel(logits.size(), eps=eps, out=logits.data.new())
y = logits + Variable(gumbel_noise)
#y = F.log_softmax(y.view(-1, logits.size(-1)) / tau)
y = F.softmax(y.view(-1, logits.size(-1)) / tau)
return y.view_as(logits)
def gumbel_softmax(logits, tau=1, hard=False, eps=1e-10):
"""
Sample from the Gumbel-Softmax distribution and optionally discretize.
Args:
logits: [batch_size, n_class] unnormalized log-probs
tau: non-negative scalar temperature
hard: if True, take argmax, but differentiate w.r.t. soft sample y
Returns:
[batch_size, n_class] sample from the Gumbel-Softmax distribution.
If hard=True, then the returned sample will be one-hot, otherwise it will
be a probability distribution that sums to 1 across classes
Constraints:
- this implementation only works on batch_size x num_features tensor for now
based on
https://github.com/ericjang/gumbel-softmax/blob/3c8584924603869e90ca74ac20a6a03d99a91ef9/Categorical%20VAE.ipynb ,
(MIT license)
"""
shape = logits.size()
assert len(shape) == 2
y_soft = gumbel_softmax_sample(logits, tau=tau, eps=eps)
if hard:
_, k = y_soft.data.max(-1)
# this bit is based on
# https://discuss.pytorch.org/t/stop-gradients-for-st-gumbel-softmax/530/5
y_hard = logits.data.new(*shape).zero_().scatter_(-1, k.view(-1, 1), 1.0)
# this cool bit of code achieves two things:
# - makes the output value exactly one-hot (since we add then
# subtract y_soft value)
# - makes the gradient equal to y_soft gradient (since we strip
# all other gradients)
y = Variable(y_hard - y_soft.data) + y_soft
else:
y = y_soft
return y
class FactorizedEmbeddingsInput(nn.Module):
def __init__(self, emb_table, vocab_map):
'''
emb_table: numpy array (V x E)
vocab_map: {word -> [indices]}
'''
super().__init__()
self.emb_tables = nn.Embedding(*emb_table.shape)
self.emb_tables.weight.data.copy_(torch.from_numpy(emb_table))
self.vocab_map = vocab_map
self.index_map = torch.LongTensor(list(vocab_map.values()))
def forward(self, indices):
# B x S -> BS x M
exp_indices = self.index_map[indices.data.view(-1)]
# exp_indices = exp_indices.view(indices.size(0), indices.size(1), -1)
# BS x M -> BS x M x E
exp_embs = self.emb_tables(Variable(exp_indices))
# BS x M x E -> BS x E
exp_embs = exp_embs.sum(-2)
# BS x E -> B x S x E
exp_embs = exp_embs.view(indices.size(0), indices.size(1), -1)
return exp_embs
@classmethod
def load_hdf5(cls, h5_file):
print('Loading compressed embeddings in %s' % h5_file)
f = h5py.File(h5_file, 'r')
# embeddings
embs = np.array(f['embeddings'])
words = list(f['vocab'])
indices = list(f['indices'])
# print(embs.dtype, words, indices)
vocab_map = {k: v.tolist() for k, v in zip(words, indices)}
return cls(embs, vocab_map)
class FactorizedEmbeddingsOutput(nn.Module):
def __init__(self, emb_table, vocab_map):
'''
emb_table: numpy array (V x E)
vocab_map: {word -> [indices]}
'''
super().__init__()
self.linear = nn.Linear(*emb_table.shape, bias=False)
self.linear.weight.data.copy_(torch.from_numpy(emb_table))
self.vocab_map = vocab_map
self.index_map = torch.LongTensor(list(vocab_map.values()))
def forward(self, x):
# B x E -> B x MK
mk_scores = self.linear(x)
# B x MK -> B x V
v_size = self.index_map.size(0)
v_scores = [mk_scores[:, self.index_map[i]].sum(1)
for i in range(v_size)]
return torch.stack(v_scores, 1)
@classmethod
def load_hdf5(cls, h5_file):
print('Loading compressed embeddings in %s' % h5_file)
f = h5py.File(h5_file, 'r')
# embeddings
embs = np.array(f['embeddings'])
words = list(f['vocab'])
indices = list(f['indices'])
# print(embs.dtype, words, indices)
vocab_map = {k: v.tolist() for k, v in zip(words, indices)}
return cls(embs, vocab_map)
class EmbeddingCompressor(nn.Module):
def __init__(self, emb_size, n_tables, n_codes, temp=1, hard=True):
"""
emb_size: (E) embedding size (both in input and output)
n_tables: (M) number of embedding tables
n_codes: (K) number of codes in each table
temp: temperature of gumbel-softmax
hard: switch between hard and soft gumbel-softmax
"""
super().__init__()
self.emb_size = emb_size
self.n_codes = n_codes
self.n_tables = n_tables
self.temp = temp
self.hard = hard
mk_half = n_tables * n_codes // 2
self.linear1 = nn.Linear(emb_size, mk_half)
self.linear2 = nn.Linear(mk_half, n_tables * n_codes)
self.emb_tables = nn.Linear(n_tables * n_codes, emb_size, bias=False)
def get_indices(self, x):
"""Given a batch of embeddings of shape B x E
returns a list-of-lists of non-zero indices, one per row
"""
# B x E -> B x MK
one_hot = self.encode(x)
return [torch.nonzero(one_hot[i]).squeeze().cpu().tolist()
for i in range(one_hot.size(0))]
def encode(self, x):
"""Given a batch of embeddings of shape B x E
returns a batch of one-hot embeddings of shape B x M x K
"""
# B x E -> B x MK/2
h = nn.Tanh()(self.linear1(x))
# B x MK/2 -> B x MK
a = nn.functional.softplus(self.linear2(h))
# B x MK -> BM x K
a = a.view(-1, self.n_codes)
# BM x K -> BM x K
d = gumbel_softmax(a, tau=self.temp, hard=self.hard)
# BM x K -> B x MK
return d.view(-1, self.n_tables * self.n_codes)
def forward(self, x):
# B x E -> B x MK
one_hot = self.encode(x)
# B x MK -> B x E
return self.emb_tables(one_hot)
<file_sep>import math
import torch
import numpy as np
import h5py
from collections import OrderedDict
def load_embeddings_txt(path, max=None, vsize=0):
train = OrderedDict()
valid = OrderedDict()
with open(path, 'rt', encoding='utf-8') as ef:
for i, line in enumerate(ef):
if i >= max+vsize:
break
tokens = line.split()
word = tokens[0]
# 1 x EmbSize
vector = np.array(tokens[1:], dtype=np.float32)[None, :]
if i < max:
train[word] = vector
if i > max:
valid[word] = vector
return train, valid
def make_vocab(word2vector):
if not word2vector:
return None
idx2word = []
word2idx = {}
all_vars = []
for word, vector in word2vector.items():
word2idx[word] = len(idx2word)
idx2word.append(word)
all_vars.append(torch.from_numpy(vector))
embeddings = torch.cat(all_vars, 0)
embeddings = torch.autograd.Variable(embeddings, requires_grad=False)
return dict(idx2word=idx2word,
word2idx=word2idx,
embeddings=embeddings,
n_embs=embeddings.size(0),
emb_size=embeddings.size(1))
def print_compression_stats(vocab, n_tables, n_codes):
n_words = vocab['n_embs']
emb_size = vocab['emb_size']
orig_mb = n_words * emb_size * 4 / 2 ** 20
comp_mb = n_tables * n_codes * emb_size * 4 / 2 ** 20
ratio = - 100 * (orig_mb - comp_mb) / orig_mb
print('[ORIG] {:4} words with embedding size {:3}: {:3.3} MB'.format(n_words, emb_size, orig_mb))
print('[COMP] {:4} words ({:2} tables, {:3} codes): {:3.3} MB ({:.2f}%)'.format(n_words, n_tables, n_codes, comp_mb, ratio))
def check_training(old_params, new_params):
if old_params is None:
return
old_sums = [p.sum().data[0] for p in old_params]
new_sums = [p.sum().data[0] for p in new_params]
for old, new in zip(old_sums, new_sums):
if old == new:
print(old_sums)
print(new_sums)
print('WARNING: some parameter did not seem to change!')
def euclidian_dist(x, y):
return math.sqrt((x - y).pow(2).sum())
def dump_reconstructed_embeddings(out_file, model, emb_dict):
print('Saving reconstructed emb_tables for train set in %s' % out_file)
with open(out_file, 'wt') as f:
for word, emb in emb_dict.items():
#print(word)
emb = torch.autograd.Variable(torch.from_numpy(emb))
emb_comp = model(emb).squeeze().cpu().data.numpy().tolist()
for x in emb_comp:
word += ' ' + str(x)
f.write(word + '\n')
def save_hdf5(out_file, model, emb_dict):
print('Saving compressed embedding in %s' % out_file)
# embeddings
f = h5py.File(h5_file, 'w')
embs = model.emb_tables.weight.cpu().data.numpy()
f.create_dataset('embeddings', data=embs)
# words
words = list(emb_dict.keys)
# indices
def load_hdf5(h5_file):
print('Loading compressed embeddings in %s' % h5_file)
f = h5py.File(h5_file, 'r')
# embeddings
embs = np.array(f['embeddings'])
words = list(f['vocab'])
indices = list(f['indices'])
#print(embs.dtype, words, indices)
vocab_map = {k: v.tolist() for k, v in zip(words, indices)}
return embs, vocab_map
def load_artichoke(h5_file):
print('Loading Artichoke file: %s' % h5_file)
f = h5py.File(h5_file, 'r')
words = list(f['/data/vocab/7fd01463c400/values'])
embs = f['/data/parameters/embedding/W']
embs = np.split(embs, embs.shape[0])
train_d = {k: v for k, v in zip(words, embs)}
#print(words)
f.close()
return train_d, None
def save_artichoke(h5_file, model, emb_dict):
print('Saving Artichoke file: %s' % h5_file)
f = h5py.File(h5_file, 'r+')
embs = []
for word, emb in emb_dict.items():
# print(word)
emb = torch.autograd.Variable(torch.from_numpy(emb))
emb_comp = model(emb).squeeze().cpu().data.numpy().tolist()
embs.append(emb_comp)
f_embs = f['/data/parameters/embedding/W']
f_embs[...] = embs
f.close()
<file_sep>import argparse
import math
import os
import torch
from torch import nn
from time import time
from embzip.data import load_embeddings_txt, make_vocab, print_compression_stats, euclidian_dist, check_training, dump_reconstructed_embeddings
from embzip.model import EmbeddingCompressor
if __name__ == '__main__':
parser = argparse.ArgumentParser(
formatter_class=argparse.ArgumentDefaultsHelpFormatter)
parser.add_argument('--path',
required=True,
help='Path of embedding file')
parser.add_argument('--n_embs',
type=int,
default=75000,
help='Number of emb_tables to consider')
parser.add_argument('--n_tables',
type=int,
required=True,
help='Number of embedding tables')
parser.add_argument('--n_codes',
type=int,
required=True,
help='Number of emb_tables in each table')
parser.add_argument('--samples',
type=int,
default=int(2e7),
help='Number of embeddings to train')
parser.add_argument('--report_every',
type=int,
default=1000,
help='Reporting interval in batches')
parser.add_argument('--batch_size',
type=int,
default=128,
help='Number of emb_tables in each batch')
parser.add_argument('--lr',
type=float,
default=0.0001,
help='Learning rate for optimizer')
parser.add_argument('--temp',
type=float,
default=1,
help='Gumbel-Softmax temperature')
parser.add_argument('--valid',
type=int,
default=1000,
help='Number of emb_tables for validation')
parser.add_argument('--cuda',
action='store_true',
help='Run on GPU')
parser.add_argument('--hard',
action='store_true',
help='Use hard gumbel softmax')
args = parser.parse_args()
# load emb_tables
train_g, valid_g = load_embeddings_txt(args.path, args.n_embs, args.valid)
train_vocab = make_vocab(train_g)
valid_vocab = make_vocab(valid_g) if valid_g else make_vocab(train_g)
# stats
print_compression_stats(train_vocab, args.n_tables, args.n_codes)
# model
ec = EmbeddingCompressor(train_vocab['emb_size'], args.n_tables, args.n_codes, args.temp, args.hard)
# optimizer
optim = torch.optim.Adam(ec.parameters(), lr=args.lr)
# criterion
criterion = torch.nn.MSELoss(size_average=True)
losses = []
old_params = None
print('[CUDA]', args.cuda)
if args.cuda:
ec.cuda()
ec = nn.DataParallel(ec)
criterion.cuda()
samples = 0
try:
while samples < args.samples:
# shuffle emb_tables
embeddings = train_vocab['embeddings'][torch.randperm(train_vocab['n_embs'])]
# train
for k in range(0, train_vocab['n_embs'], args.batch_size):
t0 = time()
samples += args.batch_size
batch = embeddings[k:k+args.batch_size]
if args.cuda:
batch = batch.cuda()
y_pred = ec(batch)
loss = criterion(y_pred, batch)
losses.append(loss.data[0])
optim.zero_grad()
loss.backward()
optim.step()
t1 = args.batch_size * 1000 * (time() - t0)
if samples % args.report_every == 0:
# check validation set and report
avg_train_loss = sum(losses) / len(losses)
losses = []
# validate
v_batch = valid_vocab['embeddings']
if args.cuda:
v_batch = v_batch.cuda()
v_y = ec(v_batch)
v_loss = criterion(v_y, v_batch)
v_loss = v_loss.data[0]
avg_euc = sum([euclidian_dist(v_y.data[i], v_batch.data[i]) for i in range(v_y.size(0))]) / v_y.size(0)
print('[%d] train: %.5f | valid: %.5f | euc_dist: %.2f | %.2f emb/sec' % (samples, avg_train_loss, v_loss, avg_euc, t1))
#print([p.sum().data[0] for p in ec.parameters()])
except KeyboardInterrupt:
print('Training stopped!')
# dump full size reconstructed embeddings to file
dump_reconstructed_embeddings(args.path + '.comp', ec, train_g)
# save compressed embeddings in hdf5 format
#:save_hdf5(args.path + '.h5;', ec, train_g)
<file_sep># MAC
http://download.pytorch.org/whl/torch-0.2.0.post3-cp36-cp36m-macosx_10_7_x86_64.whl
# LINUX
# http://download.pytorch.org/whl/cu80/torch-0.2.0.post3-cp36-cp36m-manylinux1_x86_64.whl
torchvision
jupyter
pandas
sklearn
matplotlib
psutil
scipy
scikit-learn
deap
update_checker
tqdm
stopit
# xgboost
scikit-mdr
skrebate
tpot
h5py
nose
parametrized
| bf445793d18e2328eb8725738921984fbe783240 | [
"Python",
"Text"
] | 5 | Python | ReiiSky/embzip | 144d0674e0fc174fa14d58fa8125d548ba6fca25 | 5945fa06bbeaddbc00bca88421e233bfe2671525 | |
refs/heads/master | <file_sep>import { HttpClient } from '@angular/common/http';
import { Injectable } from '@angular/core';
import { environment } from 'src/environments/environment';
import { PersonSummary } from './personSummary.model';
@Injectable({
providedIn: 'root'
})
export class CommonService {
personalDetails: any;
professionalDetails: any;
personalSummary: any;
summary: PersonSummary = new PersonSummary();
constructor(private http: HttpClient) { }
postData(personalDetails: any, professionalDetails: any) {
var url = environment.baseRepoUrl;
this.summary.personalData = personalDetails;
this.summary.professionalData = professionalDetails;
this.http.post(url, this.summary).subscribe(
result => {
console.log(result)
}
)
}
}
<file_sep>
export class PersonSummary {
public personalData : any;
public professionalData : any;
} | 45f63405e21e0adfd248cae01703f78f93f4ae20 | [
"TypeScript"
] | 2 | TypeScript | Mahesh3677/Angular-Lazy-Loading-main | 021bfc42f32b06a23a9ccf856947ad32253d00fa | bb0e0dd69953c99629e72e5bb2661b04094a43bd | |
refs/heads/master | <repo_name>amusements/great_shows<file_sep>/py_03_for_else/README.md
[參考網站](https://docs.python.org/3/tutorial/controlflow.html#break-and-continue-statements-and-else-clauses-on-loops)
1. Track & pull branch feature/for_else
2. Copy sample_file.py under the folder py_03_for_else and named as [yourname]_re_rule.py
3. Finish 3 excercises and match the expected output in file.
4. Push/Submit your code to github
<file_sep>/py_03_for_else/sample.py
big_box = ["Orchild", "Mickey Mouse", "Orange", "Cat", "Cow", "Lemon Tree", "Red Pepper"]
# TODO: Using for-loop & continue to add water on plants, but not animal
# OUTPUT:
# Orchild watered.
# Orange waterd.
# Lemon Tree watered.
# Red Pepper waterd.
# TODO: Using for-loop & break to feed Cow only
# OUTPUT:
# Cow feeded.
# TODO: Using for-loop & else to wash a car
# OUTPUT:
# No car to wash.
<file_sep>/py_0501_list_stack/linda.py
# Q1: Define a function which can generate and print a list where the values are square of numbers between 1 and 20. Then the function needs to print the first 5 elements in the list.
# Hint:
# Use range() for loops.
# Use list.append() to add values into a list.
# Use [n1:n2] to slice a list
# OUTPUT:
# 1
# 4
# 9
# 16
# 25
squares = []
for x in range (1,21):
squares.append(x**2)
print (squares[0:5])
# Q2: Base on Q1, please print the 11th element.
# Hint:
# Use list.index()
print (squares[11])
# Q3: Base on Q1, reverse list and pop out the top element and print out.
# Hint:
# Use list.reserse() and list.pop
squares.reverse()
#print (squares)
squares.pop(0)
print (squares)
# Q4: Base on Q1, please insert "I am rock" into 13th position, then print out each element in list.
# Hint:
# Use list.insert() and for loop
squares.insert(13,"I am rock")
print (squares)
# Q5: Base on Q4, please remove "I am rock", then print out each element in list.
# Hint:
# Use list.remove()
squares.remove("I am rock")
print (squares)
<file_sep>/py_01_search_text/rika_search.py
inputText = input("Please enter keyword for search: ")
file = open("article.txt", "r")
fileContent = file.readlines()
file.close()
inputTextLower = inputText.lower()
matchedCount = 0;
for line in fileContent:
for word in line.split():
wordLower = word.lower()
matchedCount += wordLower.count(inputTextLower)
print("Keyword {}: We have found {} matched in article".format(inputText, matchedCount))
<file_sep>/linda_practice/linda_re_rule.py
big_box = ["Orchild", "Mickey Mouse", "Orange", "Cat", "Cow", "Leon Tree", "Red Pepper"]
#plants = ["Orchild", "Orange", "Leon Tree", "Red Pepper"]
animals = ["Mickey Mouse", "Cat", "Cow"]
for obj in big_box:
if (obj != animals):
print (obj,"watered")
continue
for obj in big_box:
if (obj == "Cow"):
print (obj,"feeded")
break
for obj in big_box:
if (obj == "car"):
print (obj,"is a car")
break
else:
print ("No car to wash")
<file_sep>/py_0501_list_stack/lily.py
#!/Users/yaliyang/anaconda3/bin/python3
square_list = []
for i in range (1, 21):
square_list.append(i**2)
print (square_list [0:5])
print (square_list [11])
square_list.reverse()
square_list.pop(0)
print (square_list)
square_list.reverse()
square_list.append(400)
print (square_list)
square_list.insert(12, "I am rock")
print (square_list)
square_list.remove("I am rock")
print (square_list)
<file_sep>/py_0501_list_stack/daniel.py
result = []
for i in range (1,21):
a = i**2
result.append(a)
##Q1
print(result[:5])
##Q2
print(result[10])
##Q3
result.reverse()
print(result.pop())
##Q4
result.insert(12, "I am rock")
print(result)
##Q5
result.remove("I am rock")
print(result)
<file_sep>/py_06_api_practice/daniel.py
import re
import json
import urllib.request, urllib.parse, urllib.error
from datetime import datetime
while True:
a = "a. 戲劇開始日期與結束日期"
b = "b. 表演地點"
search = "請選擇使用何種方式搜尋 (a,b): "
print(a + "\n" + b)
searchtype = input(search)
autotime = "00:00:00"
get_loc = ""
dt_start_time = ""
dt_end_time = ""
tw_city =("臺北市","台北市","新北市","桃園市","臺中市","臺南市","高雄市","基隆市","新竹市",
"嘉義市","新竹縣","苗栗縣","彰化縣","南投縣","雲林縣","嘉義縣","屏東縣","宜蘭縣","花蓮縣","臺東縣","澎湖縣")
if searchtype == "a":
while True:
try:
get_st_dt = str(input("請輸入欲查詢之開始時間 (YYYY/MM/DD): "))
dt_start = datetime.strptime(get_st_dt, '%Y/%m/%d')
dt_start_time = "{} {}" .format(get_st_dt, autotime)
break
except ValueError:
print ("開始時間錯誤,請重新輸入一次")
while True:
try:
get_end_dt = str(input("請輸入欲查詢之結束時間 (YYYY/MM/DD): "))
dt_end = str(datetime.strptime(get_end_dt, '%Y/%m/%d'))
dt_end_time = "{} {}" .format(get_end_dt, autotime)
break
except ValueError:
print ("結束時間錯誤,請重新輸入一次")
if searchtype == "b":
while True:
get_loc = input("請輸入欲查詢之表演地點(xx 縣 / 市): ")
if get_loc not in tw_city:
print ("沒這種縣市,當什麼假外國人?")
else:
break
break
else:
print("你選了什麼鳥? 重新輸入不然不讓你離開")
url = 'https://cloud.culture.tw/frontsite/trans/SearchShowAction.do?method=doFindTypeJ&category=2'
data = urllib.request.urlopen(url).read().decode()
count = 0
try:
js = json.loads(data)
except:
js = None
for item in js:
for value in item['showInfo']:
if dt_start_time <= value['time'] <= dt_end_time or get_loc in value['location']:
title = item['title']
time = value['time']
location = value['location']
print('戲劇名稱:' ,title, '表演地點:',location, '表演時間', time)
count +=1
else:
print("搜尋完畢,您的結果有",count,"筆")
<file_sep>/py_02_regular_expression/README.md
[參考網站 1](http://larry850806.github.io/2016/06/23/regex/)
[參考網站 2](https://docs.python.org/3/library/re.html)
1. Track & pull branch feature/regular_expression
2. Create python file under the folder py_02_regular_expression and named as [yourname]_re_rule.py
3. Execute [yourname]_re_rule.py, then prompt a dialog to input the keyword in order to rule the keyword category. (There are 3 categories in total, plz check out the samples.)
4. Print the keyword's category
5. Push/Submit your code to github
Extra bonus (I will value the bonus for whom did it right):
6. Validate the input tw id is effective or not
Output sample 1:
Keyword XXX is a email
Output sample 2:
Keyword XXX is a mobile phone number
Output sample 3:
Keyword XXX is a TW id
<file_sep>/py_02_regular_expression/yiwen_re_rule.py
import re
# DEFINE
inputText = input("Please enter keyword: ")
mailRegular = '^[0-9a-z._+-]*@[a-z0-9]+(\.[a-z0-9-]+)*(\.[a-z]{2,4})$'
phoneRegular = '^09[0-9]{8}$'
twIdRegular = '^[A-Z]{1}[0-9]{9}$'
idVerifyDictionary = {"A": "10", "B": "11", "C": "12",
"D": "13", "E": "14", "F": "15",
"G": "16", "H": "17", "I": "34",
"J": "18", "K": "19", "L": "20",
"M": "21", "N": "22", "O": "35",
"P": "23", "Q": "24", "R": "25",
"S": "26", "T": "27", "U": "28",
"V": "29", "W": "32", "X": "30",
"Y": "31", "Z": "33"}
weight = [1,9,8,7,6,5,4,3,2,1,1]
# FUNCTION(s)
def is_tw_id(inStr):
head = idVerifyDictionary[inStr[0]]
body = inStr[1:10]
e = head + body
total = 0
for index, val in enumerate(weight):
total += (int(val) * int(e[index]))
if total % 10 == 0:
print("Keyword {} is a TW id".format(inStr))
else :
print("Keyword {} is not TW id".format(inStr))
# MAIN
if re.match(mailRegular, inputText) != None:
print("Keyword {} is a email".format(inputText))
elif re.match(phoneRegular, inputText) != None:
print("Keyword {} is a mobile phone number".format(inputText))
elif re.match(twIdRegular, inputText) != None:
is_tw_id(inputText)
else :
print("Keyword {} is not matched".format(inputText))
<file_sep>/py_03_for_else/lily_re_rule.py
#!/Users/yaliyang/anaconda3/bin/python3
g_box = ["Orchild", "Mickey Mouse", "Orange", "Cat", "Cow", "Lemon Tree", "Red Pepper"]
# TODO: Using for-loop & continue to add water on plants, but not animal
# OUTPUT:
# Orchild watered.
# Orange waterd.
# Lemon Tree watered.
# Red Pepper waterd.
# TODO: Using for-loop & break to feed Cow only
# OUTPUT:
# Cow feeded.
# TODO: Using for-loop & else to wash a car
# OUTPUT:
# No car to wash.
#plants = {"Orchild", "Orange", "Lemon Tree", "Red Pepper"}
#animals = {"Mickey Mouse", "Cat", "Cow"}
c_box = {
"Orchild": "plant", "Mickey Mouse": "animal", "Orange": "plant", "Cat": "animal",
"Cow": "animal", "Lemon Tree": "plant", "Red Pepper": "plant"
}
for key, value in c_box.items():
if value == "animal":
continue
if value == "plant":
print (key, "watered")
#for i in g_box:
# if i == "Cow":
# print ("Cow feeded")
# break
#for i in g_box:
# if i == "car":
# print ("car washed")
#else:
# print ("No car to wash")
<file_sep>/py_01_search_text/daniel_search.py
#!/usr/local/bin/python3
''' ----- search practice -----'''
Keyword = input("Type Keyword: ")
k=0
with open("article.txt","r") as f:
for line in f:
words = line.split()
for i in words:
if (i.lower() ==Keyword):
k=k+1
print("We have found", k, "matched in article")<file_sep>/py_03_for_else/Daniel_re_rule.py
big_box = ["Orchild", "Mickey Mouse", "Orange", "Cat", "Cow", "Leon Tree", "Red Pepper"]
# TODO: Using for-loop & continue to add water on plants, but not animal
# OUTPUT:
# Orchild watered.
# Orange waterd.
# Leon Tree watered.
# Red Pepper waterd.
for item in big_box:
if item in ["Mickey Mouse", "Cat", "Cow"]:
continue
else:
print (item + " water")
#plants = ['Orchild', 'Orange', 'Leon Tree', 'Red Pepper']
#if item in plants:
# print (item + " water")
# TODO: Using for-loop & break to feed Cow only
# OUTPUT:
# Cow feeded.
for item in big_box:
if item == "Cow":
print(item + " feeded")
break
# TODO: Using for-loop & else to wash a car
# OUTPUT:
# No car to wash.
for item in big_box:
if item == "Car":
print("I found a car")
break
else:
print("No car to wash")<file_sep>/py_02_regular_expression/rika_re_rule.py
import re
inputText = input("Please enter keyword: ")
mailRegular = '^[0-9a-z._+-]*@[a-z0-9]+(\.[a-z0-9-]+)*(\.[a-z]{2,4})$'
phoneRegular = '^09[0-9]{8}$'
twIdRegular = '^[A-Z]{1}[0-9]{9}$'
idVerifyDictionary = {"A": 10, "B": 11, "C": 12,
"D": 13, "E": 14, "F": 15,
"G": 16, "H": 17, "I": 34,
"J": 18, "K": 19, "L": 20,
"M": 21, "N": 22, "O": 35,
"P": 23, "Q": 24, "R": 25,
"S": 26, "T": 27, "U": 28,
"V": 29, "W": 32, "X": 30,
"Y": 31, "Z": 33}
def verify_tw_id(tw_id):
strStrip = tw_id.strip()
strStripMaxIndex = len(strStrip)-1
strStripFirstWord = strStrip[0]
verifyKey = idVerifyDictionary[strStripFirstWord]
verifyInt = int(strStrip[strStripMaxIndex])
verifyInt += int(verifyKey / 10) + (verifyKey % 10) * 9
i = 8;
for x in range(1, strStripMaxIndex):
verifyInt += int(strStrip[x]) * i
i -= 1
if verifyInt % 10 == 0:
return 1
else :
return 0
if re.match(mailRegular, inputText) != None:
print("Keyword {} is a email".format(inputText))
elif re.match(phoneRegular, inputText) != None:
print("Keyword {} is a mobile phone number".format(inputText))
elif re.match(twIdRegular, inputText) != None:
if bool(verify_tw_id(inputText)):
print("Keyword {} is a TW id".format(inputText))
else :
print("Keyword {} is not TW id".format(inputText))
else :
print("Keyword {} is not matched".format(inputText))
<file_sep>/py_0501_list_stack/sample.py
# Q1: Define a function which can generate and print a list where the values are square of numbers between 1 and 20. Then the function needs to print the first 5 elements in the list.
# Hint:
# Use range() for loops.
# Use list.append() to add values into a list.
# Use [n1:n2] to slice a list
# OUTPUT:
# 1
# 4
# 9
# 16
# 25
# Q2: Base on Q1, please print the 11th element.
# Hint:
# Use list.index()
# Q3: Base on Q1, reverse list and pop out the top element and print out.
# Hint:
# Use list.reserse() and list.pop
# Q4: Base on Q1, please insert "I am rock" into 13th position, then print out each element in list.
# Hint:
# Use list.insert() and for loop
# Q5: Base on Q4, please remove "I am rock", then print out each element in list.
# Hint:
# Use list.remove()
<file_sep>/py_06_api_practice/rika.py
import re
dateRegular = '^[0-9]{4}/[0-9]{2}/[0-9]{2}$'
def check_date_format(dateStr):
if len(dateStr) == 0:
return True
if re.match(dateRegular, dateStr) == None:
print('dateRegular')
return False
dateArr = dateStr.split('/')
if dateArr[1] < '01' or dateArr[1] > '12' or dateArr[2] < '01' or dateArr[2] > '31':
print('dateArr')
return False
return True
import json
import urllib.request, urllib.parse, urllib.error
def get_josn_data(startDateStr, endDateStr, locationStr):
sourceURL = "https://cloud.culture.tw/frontsite/trans/SearchShowAction.do?method=doFindTypeJ&category=2"
source = urllib.request.urlopen(sourceURL)
decodeSource = source.read().decode()
sourceJson = json.loads(decodeSource)
dramaArr = []
for objDict in sourceJson:
for infoDict in objDict["showInfo"]:
location = infoDict["location"]
if len(locationStr) > 0 and not locationStr in location:
continue;
time = infoDict["time"]
if (len(startDate) > 0 and len(startDate) == len(endDate) and (time < startDate or time > endDate)):
continue;
elif (len(startDate) > 0 and time < startDate):
continue;
elif (len(endDate) > 0 and time > endDate):
continue;
title = objDict["title"]
locationName = infoDict["locationName"]
print("Drama Title: ",title)
print("Time: ",time)
print("Location: {0} ({1})".format(location, locationName))
dramaDic = {"title": title, "time": time, "location": location, "locationName": locationName}
dramaArr.append(dramaDic)
return dramaArr
startDate = input("Please enter start date for search(EX: 2018/01/01): ")
endDate = input("Please enter end date for search(EX: 2018/01/01): ")
location = input("Please enter location for search(EX: 國家戲劇院): ")
print('Searching..............')
errorMsg = ''
if not check_date_format(startDate) or not check_date_format(endDate):
errorMsg = "Start date or end date has wrong format!\nDate format should be like 2018/01/01"
elif len(startDate) > 0 and len(endDate) > 0 and startDate > endDate:
errorMsg = "Start date must be earlier than end date."
if len(errorMsg) > 0:
print('Error: ',errorMsg)
else:
resultArr = get_josn_data(startDate, endDate, location)
print("Total result count: {0}".format(len(resultArr)))
<file_sep>/py_06_api_practice/test.py
import datetime
import json
import urllib.request, urllib.parse, urllib.error
import sys
inputStart = input("Please enter start date for search: ")
inputEnd = input("Please enter end date for search: ")
inputLocate = input("Please enter location for search: ")
#sys.exit(inputText)
url = "https://cloud.culture.tw/frontsite/trans/SearchShowAction.do?method=doFindTypeJ&category=2"
data_str = urllib.request.urlopen(url).read().decode()
data = json.loads(data_str)
count = 0
for obj in data:
if inputEnd >= obj["startDate"] and inputStart <= obj["endDate"]:
for info in obj["showInfo"]:
if inputLocate in info["location"]:
count +=1
print(info["time"], ', ', obj["title"], ', ', info["location"])
print("Total count:", count)<file_sep>/py_06_api_practice/README.md
##[Reference Website]
https://docs.python.org/3/tutorial/datastructures.html 5.5 dictionaries, 5.6 looping
https://docs.python.org/3/library/json.html import json
https://docs.python.org/3/library/urllib.html import urllib
##Hand-in instruction
1. Pull master branch and find the new folder, `py_06_api_practice`.
2. Create your own branch named it as `[your name]_api_drama` based on master
3. Read through 'README.md' file
4. Name your homework file as `[your name].py`
5. Commit your homwork file to local branch
6. Push your branch to remote
7. Create PR and add reviewer on Github
In this Assignment, you will need to use python to extract data from JSON, one of the most popular format of API.
##Deliverable:
Use python to call API and display the following details:
1. Drama Title
2. Location
3. Performance time (greater than 2018/01/01 00:00:00)
4. Total count of dramas with different time (same drama but different time are different counts)
##Materials:
API: https://cloud.culture.tw/frontsite/trans/SearchShowAction.do?method=doFindTypeJ&category=2 (Highly recommend to install Chrome extension JSONView to have a better look at data structure)
##Time estimation: 0.5 to 1.5 hours
##Limitation:
None, do whatever you like as long as you can extract and display the deliverable.
##!!CAUTION!! Hard-code may serious affect the performance and get BOOs from other people!
##Assignment instruction
You may found helpful `Sample code below but suggest follow the step and try it first`
1. Import libraries json urllib mentioned at reference
2. Open,read and decode the API url, you may use print to test and found something like
```
0:00"}],"showUnit":"","discountInfo":"","descriptionFilterHtml":"","imageUrl":"","masterUnit":[],"subUnit":[],"supportUnit":[],"otherUnit":[],"webSales":"https://www.accupass.com/event/1806181412251090157280","sourceWebPromote":"","comment":"","editModifyDate":"","sourceWebName":"花蓮縣文化局","startDate":"2018/08/22","endDate":"2018/08/22","hitRate":0},{"version":"1.4","UID":"5b55bd96d083a3989c55aa14","title":"107年花蓮漫畫夏令營","category":"2","showInfo":[{"time":"2018/08/02 00:00:00","location":"花蓮市中山路71號","locationName":"花蓮鐵道文化園區一館中山堂","onSales":"N","price":"","latitude":null,"longitude":null,"endTime":"2018/08/21 00:00:00"}],"showUnit":"","discountInfo":"","descriptionFilterHtml":"","imageUrl":"","masterUnit":[],"subUnit":[],"supportUnit":[],"otherUnit":[],"webSales":"https://www.facebook.com/fishanima/","sourceWebPromote":"","comment":"","editModifyDate":"","sourceWebName":"花蓮縣文化局","startDate":"2018/08/02","endDate":"2018/08/21","hitRate":0}]
```
3. Extract JSON object by using json.loads function
4. Write loop to get the target details and counts `Hint: Review the 5.5 and 5.6`
```Sample Code, please replace 'something'```
import json
import urllib.request, urllib.parse, urllib.error
urllib.request.urlopen('something')
'something'.read().decode()
json.loads('something')
<file_sep>/py_06_api_practice/lily.py
#!/Users/yaliyang/anaconda3/bin/python3
import re
import json
import urllib.request, urllib.parse, urllib.error
drama= urllib.request.urlopen('https://cloud.culture.tw/frontsite/trans/SearchShowAction.do?method=doFindTypeJ&category=2 ')
drama_list= drama.read().decode()
total_list=json.loads(drama_list)
city = input("City/County:")
start= input("Start date(0000/00/00 00:00:00):")
end= input("End date(0000/00/00 00:00:00):")
theater = input("Theater:")
if len(city) ==0:
print("You have to fill all the information needed!")
elif len(start) ==0:
print("You have to fill all the information needed")
elif len(end) ==0:
print("You have to fill all the information needed")
elif len(theater) ==0:
print("You have to fill all the information needed")
for dramadict in total_list:
for infodict in dramadict["showInfo"]:
title = dramadict["title"]
time = infodict["time"]
location = infodict["location"]
locationName = infodict["locationName"]
price = infodict["price"]
# recity = re.sub("台", "臺", str(infodict["location"]))
if (city in infodict["location"]) and (start <= infodict["time"] <= end) and (theater in infodict["locationName"]):
print ("Drama Title:", title)
print ("Performance time:", time)
print ("Theater: {0} ({1})".format(locationName, location))
print ("Price:", price)
<file_sep>/py_01_search_text/linda_search.py
word = format(input('Input yout word: ')).lower()
with open('article.txt','r') as f:
for line in f:
if word in line.lower():
print ("keyword",(word),":We have found",(sum(line.count(word) for line in f)),"matched in article")<file_sep>/README.md
# great_shows
Collection of performance in Taiwan with nice data format.
<file_sep>/py_02_regular_expression/daniel_re_rule.py
#!/usr/local/bin/python3
import re
Keyword = input("Please tpye the keyword: ")
Email = re.match("[^@]+@[^@]+\.[^@]+",Keyword)
Phone = re.match("^09\d{8}",Keyword)
TWID = re.match("^[A-Z][12][0-9]{8}$",Keyword)
def letter(x):
return{
"A":10,
"B":11,
"C":12,
"D":13,
"E":14,
"F":15,
"G":16,
"H":17,
"I":34,
"J":18,
"K":19,
"L":20,
"M":21,
"N":22,
"O":35,
"P":23,
"Q":24,
"R":25,
"S":26,
"T":27,
"U":28,
"V":29,
"W":32,
"X":30,
"Y":31,
"Z":33,
}[x]
def TWID(x):
letter_str = int(str(letter(Keyword[0]))+(Keyword[1:])) #Change letter to number and merge w/ rest ID number
letter_list = list(map(int,str(letter_str))) #Convert letter_str to list for calculation
num = [1,9,8,7,6,5,4,3,2,1,1] #ID check rule
verify = list(map(lambda x,y:x*y,letter_list,num)) #multiply ID w/ checkrule
if sum(verify)%10 == 0:
print (Keyword, "is a valid TWID")
else:
print(Keyword, "is not a valid TWID")
if Email:
print(Keyword, "is an email address")
elif Phone:
print(Keyword, "is an mobile phone number")
elif TWID:
TWID(Keyword)
elif ValueError:
print("Can't verify the Keyword")
'''
#--------list multiply test------
a = [1,2,3]
b = [4,5,6]
c = list(map(lambda x,y:x*y,a,b))
print(reduce(lambda x,y:x*y,c))
'''
<file_sep>/py_0501_list_stack/assignment.md
[參考網站](https://docs.python.org/3/tutorial/datastructures.html#using-lists-as-stacks)
1. Pull master branch then you will see the new folder, `py_0501_list_stack`.
2. Create your own branch named it as `[your name]_list_stack` based on master
3. Read through 'assignment.md' file
4. Name your homework file as `[your name].py`
5. Commit your homwork file to local branch
6. Push your branch to remote
7. Create PR and add reviewer on Github
#### Q1: Define a function which can generate and print a list where the values are square of numbers between 1 and 20. Then the function needs to print the first 5 elements in the list.
```
* Hint:
* Use range() for loops.
* Use list.append() to add values into a list.
* Use [n1:n2] to slice a list
```
#### Q2: Base on Q1, please print the 11th element.
* Hint: Use list.index()
#### Q3: Base on Q1, reverse list and pop out the top element and print out.
* Hint: Use list.reserse() and list.pop
#### Q4: Base on Q1, please insert "I am rock" into 13th position, then print out each element in list.
* Hint: Use list.insert() and for loop
#### Q5: Base on Q4, please remove "I am rock", then print out each element in list.
* Hint: Use list.remove()
<file_sep>/py_03_for_else/rika_re_rule.py
big_box = ["Orchild", "Mickey Mouse", "Orange", "Cat", "Cow", "Lemon Tree", "Red Pepper"]
animal = ["Mickey Mouse", "Cat", "Cow"]
# TODO: Using for-loop & continue to add water on plants, but not animal
# OUTPUT:
# Orchild watered.
# Orange waterd.
# Lemon Tree watered.
# Red Pepper waterd.
def add_water_on_plants():
for thing in big_box:
if thing in animal:
continue
print(thing)
# TODO: Using for-loop & break to feed Cow only
# OUTPUT:
# Cow feeded.
def feed_cow():
for thing in big_box:
if thing == "Cow":
print(thing+" feeded")
break
# TODO: Using for-loop & else to wash a car
# OUTPUT:
# No car to wash.
def wash_car():
for thing in big_box:
if thing == "Car":
break
else:
print("No car to wash")
print("[Practice 1] Using for-loop & continue to add water on plants, but not animal")
add_water_on_plants()
print("\n[Practice 2] Using for-loop & break to feed Cow only")
feed_cow()
print("\n[Practice 3] Using for-loop & else to wash a car")
wash_car()
<file_sep>/py_02_regular_expression/linda_re_rule.py
word = input('Input your word:').strip()
import re
phoneRegular = re.compile('^09\d{8}$')
phoneMatch = phoneRegular.match(word)
idRegular = re.compile('^[A-Z]\d{9}$')
idMatch = idRegular.match(word)
emailRegular = re.compile('^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+$')
emailMatch = emailRegular.match(word)
if phoneMatch:
print ("keyword",word,"is a mobile phone number.")
elif idMatch:
print ("keyword",word,"is a id.")
elif emailMatch:
print ("keyword",word,"is a email.")
else:
print ("error!")<file_sep>/py_01_search_text/lily_search.py
#!/Users/yaliyang/anaconda3/bin/python3
file = open('article.txt','r')
word = file.read()
file.close
show = input("Keyword : " )
output = word.count(show)
print("Keyword", show, ":", "We have found", output, " matched in the article")
<file_sep>/py_06_api_practice/linda.py
import re
import datetime
import urllib.request
import json
StartDate = input('輸入開始時間:')
EndDate = input('輸入結束時間:')
Location = input('輸入地點:')
TimeRegular = re.compile(('[0-1][0-9]|2[0-3])\/[0-5][0-9]\/[0-5][0-9]')
TimeMatch = TimeRegular.search(StartDate,EndDate)
if TimeMatch:
return True
else:
print ("error!")
response = urllib.request.urlopen('https://cloud.culture.tw/frontsite/trans/SearchShowAction.do?method=doFindTypeJ&category=2')
response = response.read().decode('utf-8')
responseJason = json.loads(response)
for dataDict in responseJason:
if EndDate >= obj["startDate"] and StartDate <= obj["endDate"] :
for infoDict in dataDict["showInfo"]:
if Location in info["location"]:
print("Drama Title: "+title)
print("Location: {0} ({1})".format(locationName, location))
print("Performance time: "+time)
else:
print("找不到資料")
<file_sep>/py_07_database_sqlite/README.md
##[Reference Website]
https://pythonspot.com/python-database-programming-sqlite-tutorial/
##Hand-in instruction
1. Pull master branch and find the new folder, `py_07_database_sqlite`.
2. Create your own branch named it as `[your name]_database_sqlite` based on master
3. Read through 'README.md' file
4. Name your homework file as `[your name].py`
5. Commit your homwork file to local branch
6. Push your branch to remote
7. Create PR and add reviewer on Github
##Materials:
API: https://cloud.culture.tw/frontsite/trans/SearchShowAction.do?method=doFindTypeJ&category=2 (Highly recommend to install Chrome extension JSONView to have a better look at data structure)
##Assignment instruction
1. Import libraries json, urllib mentioned, sqlite3, sys at reference
2. Call API and get JSON object (If you forget how to do, you can check out 'py_06_api_practice' homework.)
3. Create database named `[your name].db`
4. Create table named 'Dramas' into `[your name].db`. Table Dramas has data fields 'UID', 'Title', 'Category' and data type should the same as JSON object
5. Insert 'UID', 'title', 'category' value from JSON object to table Dramas
6. Display number of all data count from database table Dramas
<file_sep>/py_0501_list_stack/rika.py
def list_stack():
# Q1: Define a function which can generate and print a list where the values are square of numbers between 1 and 20. Then the function needs to print the first 5 elements in the list.
# Hint:
# Use range() for loops.
# Use list.append() to add values into a list.
# Use [n1:n2] to slice a list
# OUTPUT:
# 1
# 4
# 9
# 16
# 25
squares = []
for i in range(1, 21):
squares.append(i*i)
print("First 5 elements are ",squares[0:5])
# Q2: Base on Q1, please print the 11th element.
# Hint:
# Use list.index()
print("The 11th element is ",squares[11])
# Q3: Base on Q1, reverse list and pop out the top element and print out.
# Hint:
# Use list.reserse() and list.pop
squares.reverse()
print("Pop out the top element of reverse list is ",squares.pop(0))
# Q4: Base on Q1, please insert "I am rock" into 13th position, then print out each element in list.
# Hint:
# Use list.insert() and for loop
squares.insert(13, "I am rock")
print("List did insert element: ", squares)
# Q5: Base on Q4, please remove "I am rock", then print out each element in list.
# Hint:
# Use list.remove()
squares.remove("I am rock")
print("List did remove element: ", squares)
list_stack()
<file_sep>/py_06_api_practice/README2.md
1. you will use the same file as previous HW used.
2. Allow user to input start date, end date, location and display the result.
3. Diplay error msg when type in wrong information or no results.<file_sep>/py_02_regular_expression/lily_re_rule.py
#!/Users/yaliyang/anaconda3/bin/python3
import re
test_dialog = input ("Keyword : ")
emailReg = '^\w+@[A-Za-z0-9]*.[A-Za-z]{3}$'
cellphoneReg = '^09[0-9]{8}$'
IDReg = r'^[A-Z]{1}[12]{1}\d{8}$'
dic_alphabet = {'A': '10', 'B': '11', 'C': '12', 'D': '13',
'E': '14', 'F': '15', 'G': '16', 'H': '17',
'I': '34', 'J': '18', 'K': '19', 'L': '20',
'M': '21', 'N': '22', 'O': '35', 'P': '23',
'Q': '24', 'R': '25', 'S': '26', 'T': '27',
'U': '28', 'V': '29', 'W': '32', 'X': '30',
'Y': '31', 'Z': '33'}
weight = [1, 9, 8, 7, 6, 5, 4, 3, 2, 1, 1]
def is_id(inStr):
cha_first = dic_alphabet[inStr[0]]
body = inStr[1:10]
all = cha_first + body
total = 0
for index, val in enumerate(weight):
total += (int(val) * int(all[index]))
if total % 10 == 0:
print ("This is a Taiwanese Id.")
else :
print ("incorrect")
if re.match(emailReg, test_dialog) != None:
print ('Keyword', test_dialog, 'is an email')
elif re.match(cellphoneReg, test_dialog) != None:
print ('Keyword', test_dialog, 'is a cellphone number')
elif re.match(IDReg, test_dialog) != None:
is_id(test_dialog)
else:
print('Invalid')
| 478f0d052eda8bb96bd44021b999ecc2c82ad200 | [
"Markdown",
"Python"
] | 31 | Markdown | amusements/great_shows | 39f83e47028afe2f739b1c182cfc30dd9a3a1d7b | 57fbd4fa4a6ec3d5bedc29ec784f2242515a910a | |
refs/heads/master | <file_sep>#!/bin/bash
source /opt/app-root/etc/generate_container_user
set -e
# User settable environment
DOTNET_CONFIGURATION="${DOTNET_CONFIGURATION:-Release}"
DOTNET_STARTUP_PROJECT="${DOTNET_STARTUP_PROJECT:-.}"
# Private environment
DOTNET_FRAMEWORK="netcoreapp1.0"
APP_DLL_NAME="$(basename "$(realpath "${DOTNET_STARTUP_PROJECT}")").dll"
APP_DLL_DIR="${DOTNET_STARTUP_PROJECT}/bin/${DOTNET_CONFIGURATION}/${DOTNET_FRAMEWORK}/publish"
ARG_FILE="arguments.txt"
if [[ -f "${ARG_FILE}" ]]; then
echo "---> Running application with arguments from file '${ARG_FILE}' ..."
PROG_ARGS="$(cat ${ARG_FILE})"
cd "${APP_DLL_DIR}" && exec dotnet "${APP_DLL_NAME}" ${PROG_ARGS}
else
echo "---> Running application ..."
cd "${APP_DLL_DIR}" && exec dotnet "${APP_DLL_NAME}"
fi
| 4393ae83c6f3fa555b0671be2d55a9956a58bead | [
"Shell"
] | 1 | Shell | rokkanen/s2i-dotnetcore | 84a69e75a02e2de88544f3b12e7dc11784d85fc3 | e1e7a50e89776857a7e49990052e78888a7d06d5 | |
refs/heads/master | <file_sep>
export const CartReducer =(state,action) =>{
const{shoppingCart,totalPrice,qty}=state;
let product;
let index;
let updatePrice;
let updateQty;
switch(action.type){
case 'ADD_TO_CART':
const chack=shoppingCart.find(product => product.id === action.id)
if(chack){
return state;
}
else{
product =action.product;
product['qty']= 1;
updateQty= qty + 1 ;
updatePrice= totalPrice + product.price;
return{shoppingCart:[product,...shoppingCart],totalPrice: updatePrice,qty:updateQty}
}
break;
case 'INC':
product = action.cart;
product.qty = product.qty + 1;
updatePrice = totalPrice + product.price;
updateQty = qty + 1;
index= shoppingCart.findIndex(cart => cart.id === action.id)
shoppingCart[index] = product;
return{shoppingCart:[...shoppingCart] , totalPrice:updatePrice ,qty:updateQty};
break;
case 'DEC':
product = action.cart;
if(product.qty > 1){
product.qty = product.qty - 1;
updatePrice = totalPrice - product.price;
updateQty = qty - 1;
index = shoppingCart.findIndex(cart => cart.id === action.id);
shoppingCart[index] = product;
return{shoppingCart: [...shoppingCart] , totalPrice: updatePrice , qty:updateQty};
}
else{
return state;
}
break;
case 'DELETE':
const filtered = shoppingCart.filter(product => product.id !== action.id)
product = action.cart;
updateQty = qty - product.qty;
updatePrice = totalPrice - product.price * product.qty;
return{shoppingCart: [...filtered],totalPrice: updatePrice, qty:updateQty}
default:
return state;
}
}
<file_sep>import React from 'react';
const Banner = () => {
return (
<>
<div className="banner">
<header>
</header>
</div>
</>
);
}
export default Banner;
<file_sep>import React,{createContext , useState} from 'react';
import im1 from '../assets/im1.jpg';
import im2 from '../assets/im2.jpg';
import im3 from '../assets/im3.jpg';
import im4 from '../assets/im4.jpg';
import im5 from '../assets/im5.jpg';
import im6 from '../assets/im6.jpg';
import im7 from '../assets/im7.jpg';
import im8 from '../assets/im8.jpg';
export const ProductContext = createContext();
const ProductContextProvider=(props) =>{
const [products] =useState([{
id:1,name:'Bast shoe',price:299,image:im1,
},
{id:2,name:'snaker',price:1200,image:im2,status:"new"},
{id:3,name:'Chukka boot',price:899,image:im3},
{id:4,name:'Derby shoe',price:999,image:im4,status:"new"},
{id:5,name:'Diabetic shoe',price:1700,image:im5},
{id:6,name:'Dress shoe',price:1999,image:im6},
{id:7,name:'Duckbill shoe',price:500,image:im7},
{id:8,name:'Driving moccasins',price:899,image:im8, status:"new"}
]);
return(
<ProductContext.Provider value={{ products: [...products]}}>
{props.children}
</ProductContext.Provider>
);
}
export default ProductContextProvider;<file_sep>import React, { createContext,useReducer } from 'react';
import { CartReducer } from "../GobalCantext/CartReducer";
export const CartCantext= createContext();
const CartCantextProvider=(props)=>{
const [cart,dispatch]=useReducer(CartReducer,{shoppingCart:[]
,totalPrice:0,qty:0});
return(
<CartCantext.Provider value={{...cart,dispatch}}>
{props.children}
</CartCantext.Provider>
);
}
export default CartCantextProvider;<file_sep>import React, { useContext } from 'react';
import { ProductContext} from '../../GobalCantext/ProductContex';
import { CartCantext } from "../../GobalCantext/CartCantext";
import { Link } from 'react-router-dom';
const AProduct =()=>{
const {products} = useContext(ProductContext);
const {dispatch} =useContext(CartCantext);
return(
<>
<div className="container">
<div className="products">
{products.map((product) =>{
return(
<div className="product" key={product.id}>
<div className="cover">
<div className="product-image">
<Link to={`/ViewProduct/${product.id}`}>
<img className="img-fluid" src={product.image} alt="Not Found" />
</Link>
</div>
<div className="product-Details">
<div className="product-name">
{product.name}
</div>
<div className="product-price">
${product.price}.00
</div>
</div>
<div className="add-to-cart" onClick={()=>dispatch({type:'ADD_TO_CART',id:product.id,product})} >add to cart</div>
{product.status === 'hot' ? <div className='hot'>hot</div> :''}
{product.status === 'new' ? <div className='new'>new</div> : ''}
</div>
</div>
)
})}
</div>
</div>
</>
);
}
export default AProduct; | 82c414084a5df236281c4335fbaadef33f023024 | [
"JavaScript"
] | 5 | JavaScript | kapilmatre9893/Myshoes | 1392501a36b82af8e9bc709e0e1747483cd8b0d8 | 6b66890fc2abd9e5b647e23963206a145a0adb9a | |
refs/heads/master | <file_sep><?php
namespace RegistrashunSystem\Http\Controllers\Admin;
use Illuminate\Http\Request;
use RegistrashunSystem\Http\Controllers\Controller;
use RegistrashunSystem\User;
use Illuminate\Support\Facades\Auth;
use RegistrashunSystem\Role;
use RealRashid\SweetAlert\Facades\Alert;
class UserController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
return view('admin.users.index')->with('users', User::paginate(10));
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function edit($id)
{
if (Auth::user()->id == $id) {
Alert::warning('Warning Title', 'You are not allowed to edit yourself Nwanne!!');
return redirect()->route('admin.users.index');
}
return view('admin.users.edit')->with(['user' => User::find($id), 'roles' => Role::all()]);
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id)
{
if (Auth::user()->id == $id) {
Alert::warning('Kaii', 'You are not allowed to edit yourself Nwanne!!');
return redirect()->route('admin.users.index');
}
$user = User::find($id);
$user->roles()->sync($request->roles);
Alert::success('Success Title', 'User role has been updated')->position('top-left')->showConfirmButton('Oya', 'red');
return redirect()->route('admin.users.index');
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id)
{
if (Auth::user()->id == $id) {
Alert::warning('Kaii', 'You are not allowed to Delete yourself Nwanne!!');
return redirect()->route('admin.users.index');
}
$user = User::find($id);
if ($user) {
$user->roles()->detach();
$user->delete();
Alert::success('Success Title', 'User has been Deleted!!');
return redirect()->route('admin.users.index');
}
Alert::success('warning', 'This User cannot be Deleted Ogbeni!!');
return redirect()->route('admin.users.index');
}
}
<file_sep><?php
namespace RegistrashunSystem\Http\Controllers\Admin;
use Illuminate\Http\Request;
use RegistrashunSystem\Http\Controllers\Controller;
use RegistrashunSystem\User;
class ImpersonateController extends Controller
{
public function index($id)
{
$user = User::where('id', $id)->first();
if ($user) {
session()->put('impersonate', $user->id);
}
return redirect('/home');
}
public function destroy()
{
session()->forget('impersonate');
return redirect('/home');
}
}
| 600673380ddb31dbeadb60279e8966848a72477b | [
"PHP"
] | 2 | PHP | Arindo-Mudiare/laravel-5.8-user-registration-system- | db87ba780f6dd961d07ba6042dd97402272d064c | 397ca1b90654e7091cee8b7eefe9d217c0d9ea0d | |
refs/heads/master | <file_sep>#! /usr/bin/env python3
from __future__ import division
from __future__ import print_function
ls
import os, sys, inspect
cmd_subfolder = os.path.realpath(os.path.abspath(os.path.join(os.path.split(inspect.getfile(inspect.currentframe()))[0],"src")))
if cmd_subfolder not in sys.path:
sys.path.insert(0, cmd_subfolder)
from TLeCroy import *
# --------------------------------
# constants to be set by the user
# --------------------------------
channelNr = 4
traceNr = 18
#inName = "--testpulse--"
#inName = "--testpulse--autosave--"
inName = "--testpulse--autosave--segments--"
#inDir = "/Users/Lennard/Bachelor_Thesis_LF/BA_Lennard_Franz/cs137-longzylinder/"
inDir = "cs137-longzylinder/"
#inDir = "/Users/Lennard/desktop/osci/lennard/segment-flat-CeBr-CS137/"
#inDir = "/Users/Lennard/desktop/osci/lennard/segment-CeBr-cs137/"
#inDir = "/Users/Lennard/desktop/osci/lennard/cebr-big-4ch-cs137/"
#inDir = "/volumes/lennad/Lennard Franz/cs137-longzylinder-shaper/"
#inDir = "/Users/Lennard/desktop/osci/lennard/cs137-longzylinder/"
#inDir = "/Users/Lennard/desktop/osci/lennard/cs137-filter/"
#inDir = "/Users/Lennard/desktop/autosave-segments/"
#inDir = "/Users/Lennard/Desktop/osci/lennard/cebr-cs137/"
#inDir = "/home/lfranz/work/osci/lennard/segment-longcable-CeBr-CS137/"
#inDir = "/home/lfranz/work/osci/lennard/segment-flat-plexi-background/"
#inDir = "/home/lfranz/work/osci/lennard/segment-CeBr-ra226/"
#inDir = "/home/lfranz/work/osci/lennard/segment/"
#inDir = "/home/lfranz/work/autosave-segments/"
#inDir = "/ZIH.fast/projects/d-lab_data/MAPMT/2019_gsi/segment/"
#inDir = "/ZIH.fast/projects/d-lab_data/MAPMT/2019_gsi/segments-new/"
#inDir = "/ZIH.fast/projects/d-lab_data/MAPMT/2019_gsi/segments-new/Autosave-new/"
# inDir = "/ZIH.fast/projects/d-lab_data/MAPMT/2019_gsi/Autosave/"
# --------------------------------
fileName = inDir + "C" + str(channelNr).zfill(1) + inName + str(traceNr).zfill(5) + ".trc"
TLC = TLeCroy(fileName, debug=True)
TLC.PrintPrivate()
#TLC.PlotTrace(raw=False)
header = TLC.GetHeader()
x, y = TLC.GetTrace()
y = y - header['VERTICAL_OFFSET']
#y = -y
nano = 1e-9
import numpy as np
import scipy.integrate as integrate
#print(header['VERTICAL_OFFSET'])
#print(' vertical gain:', header['VERTICAL_GAIN'])
#print('----------------')
seqLength = header['WAVE_ARRAY_COUNT'] // header['SUBARRAY_COUNT']
seqFirst = header['FIRST_VALID_PNT']
print(' LENGTH', seqLength)
print(len(x)/1000,len(y)/1000)
import matplotlib.pyplot as plt
#plt.plot(x / nano, y)
idxHigh = np.zeros(header['SUBARRAY_COUNT'],dtype=np.int)
idxLow = np.zeros(header['SUBARRAY_COUNT'],dtype=np.int)
mean = np.zeros(header['SUBARRAY_COUNT'])
std = np.zeros(header['SUBARRAY_COUNT'])
area = np.zeros(header['SUBARRAY_COUNT'])
area_all = np.zeros(header['SUBARRAY_COUNT'])
A = np.zeros(header['SUBARRAY_COUNT'])
area_bool = np.zeros(header['SUBARRAY_COUNT'])
area_mean = np.zeros(header['SUBARRAY_COUNT'])
area_all = np.zeros(header['SUBARRAY_COUNT'])
for seqNr in range(1, header['SUBARRAY_COUNT'] + 1):
#bondarys of sequences
idxHigh[seqNr-1] = seqFirst +( seqLength * seqNr - 1)
idxLow[seqNr-1] = idxHigh[seqNr-1] - seqLength + 1
#finding basline
#y[...] has to be adjusted depending vertical position of the puls
from scipy.stats import norm
mean[seqNr-1], std[seqNr-1] = norm.fit(y[ idxLow[seqNr-1] : idxLow[seqNr-1] + int(0.35*seqLength)])
#print(seqNr, idxLow, idxHigh)
mean_all = sum(mean)/header['SUBARRAY_COUNT']
std_all = sum(std)/header['SUBARRAY_COUNT']
#print(mean_all,std_all)
#plt.hist(mean, bins=100)
#plt.hist(y[idxLow[seqNr-1] : idxLow[seqNr-1]+int(0.35*seqLength)])
for seqNr in range(1, header['SUBARRAY_COUNT'] + 1):
# if mean[seqNr -1] > 0.0285 and mean[seqNr - 1] < 0.0315 and np.min( y[idxLow[seqNr-1] : idxHigh[seqNr-1]]-mean[seqNr-1]) > -0.0035:
#area of the pulse
area[seqNr-1] = integrate.trapz((y[idxLow[seqNr - 1]:idxHigh[seqNr - 1]]- mean[seqNr-1]) > 2*std[seqNr-1] ,
x[idxLow[seqNr - 1]:idxHigh[seqNr - 1]]/nano)
# area_all[seqNr-1] = integrate.trapz((y[idxLow[seqNr - 1]:idxHigh[seqNr - 1]] - mean_all) > 2*std_all ,
# x[idxLow[seqNr - 1]:idxHigh[seqNr - 1]]/nano)
#boolArr = np.where((y[idxLow[seqNr - 1]:idxHigh[seqNr - 1]]- mean[seqNr-1]) > (3*std[seqNr-1]))
boolArr = (y[idxLow[seqNr - 1]:idxHigh[seqNr - 1]]- mean[seqNr-1]) > (3*std[seqNr-1])
#print((boolArr))
#area_bool[seqNr-1] = np.sum(y[boolArr[0]])*(header['HORIZ_INTERVAL']/nano) - (mean[seqNr-1])*(len(boolArr[0])*header['HORIZ_INTERVAL']/nano)
#area_bool[seqNr-1] = np.sum(y[boolArr[0]])
area_bool[seqNr-1] = np.sum((y[idxLow[seqNr - 1]:idxHigh[seqNr - 1]]*(header['HORIZ_INTERVAL']/nano)),where = boolArr) - mean[seqNr-1]*np.sum(boolArr)*header['HORIZ_INTERVAL']/nano
#print(np.trapz(y[boolArr[0]],x[boolArr[0]])/nano,area_bool[seqNr-1])
#print(np.sum(y[idxLow[seqNr-1]:idxHigh[seqNr-1]]>(mean[seqNr-1]+3*std[seqNr-1])),len(boolArr[0]))
area_bool_positive = area_bool > 0.0
#print(seqNr, idxLow, idxHigh)
#area = integrate.trapz(y[idxLow:idxHigh],x[idxLow:idxHigh]/nano)
#print(area)
if seqNr > 18 and seqNr < 20 :
#plt.plot(x[idxLow[seqNr - 1]:idxHigh[seqNr - 1]] / nano, y[idxLow[seqNr - 1]:idxHigh[seqNr - 1]]-mean[seqNr-1], linewidth=0.5, color='k')
plt.plot(x[idxLow[0]:idxHigh[0]] / nano, (y[idxLow[seqNr - 1]:idxHigh[seqNr - 1]]-mean[seqNr-1])*1000, linewidth=1, color='k')
#area_mean[seqNr-1]= mean[seqNr-1]*len(boolArr[0])*header['HORIZ_INTERVAL']/nano
area_all[seqNr-1] = np.sum((y[idxLow[seqNr - 1]:idxHigh[seqNr - 1]]*(header['HORIZ_INTERVAL']/nano)),where = boolArr)
#print(area_all[seqNr-1])
#plt.hist(area_mean)
#plt.hist(area_all)
#print(np.sum(y[idxLow[seqNr-1]:idxHigh[seqNr-1]]>(mean[seqNr-1]+3*std[seqNr-1])))
#print(area_bool)
plt.xlabel('time (ns)')
plt.ylabel('voltage (mV)')
plt.grid()
#plt.plot(x[idxLow[428]:idxHigh[428]]/nano , y[idxLow[428]:idxHigh[428]]-mean[428])
#x=np.linspace(0,1000.1e-9,num=10001,endpoint=False)
#print(x)
#plt.plot(x , y[idxLow[3]:idxHigh[3]]-mean[3])
#area_1 = integrate.trapz((y[idxLow[1]:idxHigh[1]] - mean_all) - 1*std_all, x[idxLow[1]:idxHigh[1]]/nano)
#print(area_1)
#print("mean all:",mean_all,"std all:", std_all)
#print(mean,std)
#print("-----------------------")
#print("area corr" , area)
#print("std" , std)
#print("mean" , mean)
#print("area uncorr" , #area_1)
#print("-----------------------")
plt.xlim(-50,150)
#ax1.hist(area,bins=50)
mean_E,std_E = norm.fit(area)
#print("---------------------")
#print("mean:", mean_E, "std:", std_E)
#print("---------------------")
#plt.hist(area_bool ,bins=50)
#mean_E_all, std_E_all = norm.fit(area_bool)
#print("---------------------")
#print("mean:", mean_E_all, "std:", std_E_all)
#print("---------------------")
#import tikzplotlib
#tikzplotlib.save("pulse_mit_shaper.tex")
plt.show()
<file_sep>import numpy as np
import matplotlib.pyplot as plt
#inDir = "GSI/bi207-1660mV.txt"
#inDir = "GSI/cs137-1660mV-neu.txt"
inDir = "GSI/co60-1660mV.txt"
#inDir = "test-frequenzgenerator-pos-pulse.txt"
#inDir = "GSI/cs137-noshaper-1400mV.txt"
data = np.loadtxt(inDir)
x = data[:,0]
y = data[:,3]
#a = 32.63
#b = 0.169
#x = a*np.exp(b*x)
print(x)
N_bins = 500
bin_width = int(10000/N_bins)
idxLow = np.linspace(0,9999 - (bin_width-1), N_bins, dtype = np.int)
idxHigh = np.linspace((bin_width - 1),9999, N_bins, dtype = np.int)
idxMid = np.linspace((bin_width/2 - 1),9999 - (bin_width/2) , N_bins, dtype = np.int)
bins = np.zeros(N_bins)
n = np.zeros(N_bins)
#print(idxLow)
#print(idxMid,len(idxMid))
#print(idxHigh)
for i in range(0,N_bins,1):
#print(i)
bins[i]= x[idxMid[i]]
n[i] = np.sum(y[idxLow[i]:idxHigh[i]])
#print(n)
#print(bins)
bins_new = np.zeros(10000)
n_new = np.zeros(10000)
for i in range(0,N_bins,1):
bins_new[idxLow[i] : idxHigh[i]] = x[idxMid[i]]
n_new[idxLow[i]:idxHigh[i]] = np.sum(y[idxLow[i]:idxHigh[i]])
#print(bins_new)
#print(n_new)
a = 32.63
b = 0.169
calib = a*np.exp(b*bins)
calib_bins = calib[calib<1600]
N = n[calib<1600]
#plt.plot(x,y)
n,bins,patches=plt.hist(bins, bins, weights=n, histtype = 'step', color='k')
#n,bins,patches=plt.hist(calib_bins, bins, weights=N, histtype = 'step', color='k')
from scipy.signal import find_peaks
peaks,properties = find_peaks(n,width=3,height=600, rel_height=0.7)
print("index of peaks:", peaks)
print("X Values of peaks:", x[peaks] )
print(properties)
from scipy.optimize import curve_fit
def gauss (x,a,sigma,mu):
return a*np.exp(-(x-mu)**2/(2*sigma**2))
for peakNr in range(1, len(peaks) ):
#peakNr = 1
fit_width = 0.5
x_values = bins[np.int(peaks[peakNr] - (fit_width) * properties["widths"][peakNr]) :np.int( peaks[peakNr]+ (fit_width+0.15) * (properties["widths"][peakNr])) ]
y_values = n[np.int(peaks[peakNr] - (fit_width) * properties["widths"][peakNr]) :np.int( peaks[peakNr]+ (fit_width+0.15) * (properties["widths"][peakNr])) ]
a_bound = [0, 1.1*np.max(y_values)]
sigma_bound = [0, 1]
mu_bound = [np.min(x_values), np.max(x_values)]
popt, pcov = curve_fit(gauss, x_values , y_values, bounds= ([a_bound[0],sigma_bound[0],mu_bound[0]], [a_bound[1],sigma_bound[1],mu_bound[1]] ) )
#plt.plot(x, gauss(x, *popt), label='gauss Fit', color='g')
#plt.plot((popt[2], popt[2]), (0, 1500), 'r-')
#statistic error of curve fit
perr = np.sqrt(np.diag(pcov))
#FWHM
FWHM = 2* np.sqrt(2*np.log(2)) * popt[1]
delta_FWHM = 2* np.sqrt(2*np.log(2)) * perr[1]
print("Peak Nr:" , peakNr)
print("Fit Bereich:" , mu_bound)
print("Energy:" , popt[2], "statistic error of energy:" , perr[2], "FWHM:", FWHM, "statistic error of FWHM", delta_FWHM )
for peakNr in range(1, len(peaks) ):
#peakNr = 0
fit_width = 0.1
x_values = bins[np.int(peaks[peakNr] - (fit_width) * properties["widths"][peakNr]) :np.int( peaks[peakNr]+ (fit_width+0.2) * (properties["widths"][peakNr])) ]
y_values = n[np.int(peaks[peakNr] - (fit_width) * properties["widths"][peakNr]) :np.int( peaks[peakNr]+ (fit_width+0.2) * (properties["widths"][peakNr])) ]
a_bound = [0, 1.1*np.max(y_values)]
sigma_bound = [0, 1]
mu_bound = [np.min(x_values), np.max(x_values)]
popt, pcov = curve_fit(gauss, x_values , y_values, bounds= ([a_bound[0],sigma_bound[0],mu_bound[0]], [a_bound[1],sigma_bound[1],mu_bound[1]] ) )
plt.plot(x, gauss(x, *popt), label='gauss Fit', color='g')
plt.plot((popt[2], popt[2]), (0, 1500), 'r-')
#statistic error of curve fit
perr = np.sqrt(np.diag(pcov))
#FWHM
FWHM = 2* np.sqrt(2*np.log(2)) * popt[1]
delta_FWHM = 2* np.sqrt(2*np.log(2)) * perr[1]
print("Peak Nr:" , peakNr)
print("Fit Bereich:" , mu_bound)
print("Energy:" , popt[2], "statistic error of energy:" , perr[2], "FWHM:", FWHM, "statistic error of FWHM", delta_FWHM )
xticks = np.arange(0,110,10)
plt.xticks(xticks)
plt.xlim(0,100)
#plt.legend()
plt.grid()
plt.title("Histogramm der ToT")
plt.xlabel("ToT (ns)")
plt.ylabel("Anzahl N")
plt.xlim(0,30)
#plt.ylim(0,1250)
#import tikzplotlib
#tikzplotlib.save("GSI_generator.tex")
plt.show()
# FÜr den fi der mit dem Frequenzgernator erstellten Histogramme ergibt sich eine
# FWHM von 0.25 bis 0.35 bei 1 ns ungenauigkeit des genrator
# Trailing und falling von je 2,5ns beachten
#
#
#
#
#
#
#
#
#
<file_sep>
import iotool as io
class TLeCroy:
def __init__(self, fname, debug=None, raw=None):
self._FileName = fname
if raw:
self._Raw = raw
else:
self._Raw = False
self._Info = dict()
self._Header = dict()
self._x, self._y, self._Header, self._Info = io.readTrc(self._FileName, raw = self._Raw)
if debug:
self._Debug = debug
else:
self._Debug = False
def PrintPrivate(self):
print(" *** INFO::lecroy:PrintPrivate ***")
print(" self._FileName " + str(self._FileName))
print(" self._Debug " + str(self._Debug))
print(" self._Raw " + str(self._Raw))
print(" ---")
print(" self._Header")
for item in self._Header.items():
print(item)
print(" ---")
print(" self._Info")
for item in self._Info.items():
print(item)
print(" ------------------------")
if self._Debug:
print(type(self._y[0]))
def GetTrace(self):
return self._x, self._y
def GetY(self):
return self._y
def GetHeader(self):
return self._Header
def GetInfo(self):
return self._Info
def PlotTrace(self, raw=True):
import matplotlib.pyplot as plt
if raw:
plt.plot(self._x, self._y, 'k', label=self._FileName)
plt.ylabel('amplitude (a.u.)')
plt.xlabel('time (a.u.)')
else:
milli = 1/1000.0
plt.plot(self._x, self._y / milli, 'k', label=self._FileName)
plt.ylabel('volts (mV)')
plt.xlabel('time (s)')
plt.title(self._FileName)
plt.show()
<file_sep>#! /usr/bin/env python3
from __future__ import division
from __future__ import print_function
import os, sys, inspect
cmd_subfolder = os.path.realpath(os.path.abspath(os.path.join(os.path.split(inspect.getfile(inspect.currentframe()))[0],"src")))
if cmd_subfolder not in sys.path:
sys.path.insert(0, cmd_subfolder)
from TLeCroy import * # lecroy.py
import matplotlib.pyplot as plt
from scipy.stats import norm
import numpy as np
import scipy.integrate as integrate
import matplotlib.mlab as mlab
from scipy.signal import find_peaks, peak_widths, peak_prominences
# --------------------------------
# constants to be set by the user
# --------------------------------
channelNr = 1
StartTrace = 0
StopTrace =20
#inName = "--testpulse--"
#inName = "--testpulse--autosave--"
inName = "--testpulse--autosave--segments--"
#inDir = "/volumes/lennad/segment/segment-CeBr-cs137/"
#inDir = "/volumes/lennad/<NAME>/th-zylinder/"
inDir = "/volumes/lennad/<NAME>/cs137-longzylinder-shaper/"
#inDir = "/Users/Lennard/desktop/osci/lennard/segment-flat-CeBr-CS137/"
#inDir = "/Users/Lennard/desktop/osci/lennard/cs137-longzylinder-shaper/"
#inDir = "/Users/Lennard/destop/osci/lennard/cebr-big-4ch-cs137/"
#inDir = "/Users/Lennard/desktop/osci/lennard/segment-CeBr-ra226/"
#inDir = "/Users/Lennard/desktop/osci/lennard/ra226-filter/"
#inDir = "/Users/Lennard/desktop/autosave-segments/"
#inDir = "/Users/Lennard/desktop/osci/lennard/gag-am241/"
#inDir = "/home/lfranz/work/osci/lennard/segment-longcable-CeBr-CS137/"
#inDir = "/home/lfranz/work/osci/lennard/segment-flat-CeBr-CS137/"
#inDir = "/home/lfranz/work/osci/lennard/segment-flat-plexi-background/"
#inDir = "/home/lfranz/work/osci/lennard/segment-CeBr-am241/"
#inDir = "/home/lfranz/work/osci/lennard/segment-CeBr-cs137/"
#inDir = "/home/lfranz/work/osci/lennard/segment-CeBr-ra226/"
#inDir = "/home/lfranz/work/osci/lennard/segment/"
#inDir = "/home/lfranz/work/autosave-segments/"
#inDir = "/ZIH.fast/projects/d-lab_data/MAPMT/2019_gsi/segment/"
#inDir = "/ZIH.fast/projects/d-lab_data/MAPMT/2019_gsi/segments-new/"
#inDir = "/ZIH.fast/projects/d-lab_data/MAPMT/2019_gsi/segments-new/Autosave-new/"
# inDir = "/ZIH.fast/projects/d-lab_data/MAPMT/2019_gsi/Autosave/"
# --------------------------------
import numpy as np
import scipy.integrate as integrate
#empty array for area of sequnces for every trace
A = np.empty([0])
print(inDir)
for traceNr in range(StartTrace, StopTrace + 1, 1):
print(" INFO: working on trace: ", "C"+ str(channelNr).zfill(1) + inName + str(traceNr).zfill(5) + ".trc")
fileName = inDir + "C" + str(channelNr).zfill(1) + inName + str(traceNr).zfill(5) + ".trc"
TLC = TLeCroy(fileName, debug=True)
#TLC.PrintPrivate()
# TLC.PlotTrace(raw=False)
header = TLC.GetHeader()
x, y = TLC.GetTrace()
y = y - header['VERTICAL_OFFSET']
y = -y
nano = 1e-9
#print('----------------')
#print(' vertical gain:', header['VERTICAL_GAIN'])
#print('----------------')
seqLength = header['WAVE_ARRAY_COUNT'] // header['SUBARRAY_COUNT']
seqFirst = header['FIRST_VALID_PNT']
#print(' LENGTH', seqLength)
#plt.plot(x / nano, y)
#arrays
idxHigh = np.zeros(header['SUBARRAY_COUNT'],dtype=np.int)
idxLow = np.zeros(header['SUBARRAY_COUNT'],dtype=np.int)
mean = np.zeros(header['SUBARRAY_COUNT'])
std = np.zeros(header['SUBARRAY_COUNT'])
area = np.zeros(header['SUBARRAY_COUNT'])
area_all = np.zeros(header['SUBARRAY_COUNT'])
area_all_sum = np.zeros(header['SUBARRAY_COUNT'])
area_bool =np.zeros(header['SUBARRAY_COUNT'])
tot = np.zeros(header['SUBARRAY_COUNT'])
A = np.zeros(header['SUBARRAY_COUNT'])
# determine background
for seqNr in range(1, header['SUBARRAY_COUNT'] + 1):
#bondarys of sequences
idxHigh[seqNr-1] = seqFirst +( seqLength * seqNr - 1)
idxLow[seqNr-1] = idxHigh[seqNr-1] - seqLength + 1
#finding basline
#y[...] has to be adjusted depending vertical position of the puls
mean[seqNr-1], std[seqNr-1] = norm.fit(y[ idxLow[seqNr-1] : idxLow[seqNr-1] + int(0.35*seqLength)])
mean_all = sum(mean)/header['SUBARRAY_COUNT']
std_all = sum(std)/header['SUBARRAY_COUNT']
#Histogram of values for baseline
#plt.hist(y[ idxLow[seqNr-1] : idxLow[seqNr-1] + int(0.35*seqLength)])
#determination of area of pulses
for seqNr in range(1, header['SUBARRAY_COUNT'] + 1):
# if mean[seqNr -1] > 0.0285 and mean[seqNr - 1] < 0.0315 and np.min( y[idxLow[seqNr-1] : idxHigh[seqNr-1]]-mean[seqNr-1]) > -0.0035:
#area of the pulse
#area[seqNr-1] = np.trapz((y[idxLow[seqNr - 1]:idxHigh[seqNr - 1]]- mean[seqNr-1]) > 3*std[seqNr-1] ,
#x[idxLow[seqNr - 1]:idxHigh[seqNr - 1]]/nano)
#area_all[seqNr-1] = np.sum(y[idxLow[seqNr - 1] : idxHigh[seqNr - 1]])/(nano/header['HORIZ_INTERVAL']) - ((mean_all)*seqLength*header['HORIZ_INTERVAL']/nano)
#print(area_all_sum)
#print(area_all)
#condition to differentiate values of pulse from background
#boolArr = np.where((y[idxLow[seqNr - 1]:idxHigh[seqNr - 1]]- mean[seqNr - 1]) > (3*std[seqNr -1]))
boolArr = (y[idxLow[seqNr - 1]:idxHigh[seqNr - 1]]- mean[seqNr-1]) > (3*std[seqNr-1])
#sum of all values which meet condition and scaling
#substracting of area belonging to background
#print(y[boolArr[0]] - mean[seqNr-1])
#area_bool[seqNr-1] = np.sum(y[boolArr[0]])*(header['HORIZ_INTERVAL']/nano) - (mean[seqNr-1])*(len(boolArr[0])*header['HORIZ_INTERVAL']/nano)
A[seqNr-1]= np.max(y[idxLow[seqNr - 1]:idxHigh[seqNr - 1]])
area_bool[seqNr-1] = np.sum((y[idxLow[seqNr - 1]:idxHigh[seqNr - 1]]*(header['HORIZ_INTERVAL']/nano)),where = boolArr) - mean[seqNr-1]*np.sum(boolArr)*header['HORIZ_INTERVAL']/nano
tot[seqNr-1] = (np.sum(boolArr)-1)*header['HORIZ_INTERVAL']/nano
#print(area_bool[seqNr - 1],tot[seqNr - 1] )
#plt.plot(tot,area_bool, 'bo', ms=1)
#print(A)
#E_real = np.array([569, 662,1173,1332,1063])
#E_fit = np.array([6.485, 7.2341,10.7222,11.7675,10.2165])
E_real = np.array ([662,1173,1332,569,1063])
E_fit = np.array ([0.7535521180502402,1.1657715187599953,1.273437535904615,0.701803960984309,1.1086378999695787])
from scipy import stats
slope, intercept, r_value, p_value, std_err = stats.linregress(E_fit, E_real)
print(slope)
print(intercept)
E = intercept+slope*area_bool
print(E)
plt.plot(tot,area_bool,'bo',ms=1.5,color='k', label='Daten' )
#plt.hist2d(tot,area_bool, bins= 50)
#plt.yscale('log')
#plt.xscale('log')
from scipy.optimize import curve_fit
def fit(x,a,b):
return a*np.exp(b*x)
popt, pcov = curve_fit(fit, tot ,area_bool, bounds= ([0,0],[1000,1]),)
perr = np.sqrt(np.diag(pcov))
print(popt)
print(perr)
x_fit = np.linspace(50,106,1000)
plt.plot(x_fit, fit(x_fit, *popt), label='exp. Fit', color='r')
plt.text(52,0.75, '$A(T)=a \cdot e^{(b \cdot x)}$')
plt.text(52,0.70, '$a= 0.053 \ {nVs}$')
plt.text(52,0.65, '$b= 0.027 \ ns^{-1} $')
plt.ylim(0.15,1)
plt.legend()
plt.grid()
plt.xlabel('ToT (ns)')
plt.ylabel('Pulsfläche A (nVs) ')
#import tikzplotlib
#tikzplotlib.save("area_tot.tex")
plt.show()
<file_sep>"""
Little helper function to load data from a .trc binary file.
This is the file format used by LeCroy oscilloscopes.
<NAME> 09/2015
"""
import datetime
import numpy as np
import struct
def readTrc(fName, raw=None):
"""
Reads .trc binary files from LeCroy Oscilloscopes.
Decoding is based on LECROY_2_3 template.
[More info](http://forums.ni.com/attachments/ni/60/4652/2/LeCroyWaveformTemplate_2_3.pdf)
Parameters
-----------
fName = filename of the .trc file
Returns
-----------
x: array with sample times [s],
y: array with sample values [V],
d: dictionary with metadata
<NAME> 09/2015
"""
with open(fName, "rb") as fid:
data = fid.read(50).decode()
wdOffset = data.find('WAVEDESC')
#-------------------------------
# Get binary format / endianess
#-------------------------------
if readX( fid, '?', wdOffset + 32 ): #16 or 8 bit sample format?
smplFmt = "int16"
else:
smplFmt = "int8"
if readX( fid, '?', wdOffset + 34 ): #Big or little endian?
endi = "<"
else:
endi = ">"
#---------------------------------
# Get length of blocks and arrays
#---------------------------------
lWAVE_DESCRIPTOR = readX( fid, endi+"l", wdOffset + 36 )
lUSER_TEXT = readX( fid, endi+"l", wdOffset + 40 )
lTRIGTIME_ARRAY = readX( fid, endi+"l", wdOffset + 48 )
lRIS_TIME_ARRAY = readX( fid, endi+"l", wdOffset + 52 )
lWAVE_ARRAY_1 = readX( fid, endi+"l", wdOffset + 60 )
lWAVE_ARRAY_2 = readX( fid, endi+"l", wdOffset + 64 )
#--------------------------
# Save formats and lengths
#--------------------------
info = dict()
info["wdOffset"] = wdOffset
info["smplFmt"] = smplFmt
info["endi"] = endi
info["lWAVE_DESCRIPTOR"] = lWAVE_DESCRIPTOR
info["lUSER_TEXT"] = lUSER_TEXT
info["lTRIGTIME_ARRAY"] = lTRIGTIME_ARRAY
info["lRIS_TIME_ARRAY"] = lRIS_TIME_ARRAY
info["lWAVE_ARRAY_1"] = lWAVE_ARRAY_1
info["lWAVE_ARRAY_2"] = lWAVE_ARRAY_2
#--------------------------------------
# Get Instrument info from file header
#--------------------------------------
h = dict() #Will store all the extracted Metadata
h["INSTRUMENT_NAME"] = readX( fid, "16s", wdOffset + 76 ).decode().split('\x00')[0]
h["INSTRUMENT_NUMBER"]= readX( fid, endi+"l", wdOffset + 92 )
h["TRACE_LABEL"] = readX( fid, "16s", wdOffset + 96 ).decode().split('\x00')[0]
#------------------------------------
# Get Waveform info from file header
#------------------------------------
h["WAVE_ARRAY_COUNT"] = readX( fid, endi+"l", wdOffset + 116 )
h["PNTS_PER_SCREEN"] = readX( fid, endi+"l", wdOffset + 120 )
h["FIRST_VALID_PNT"] = readX( fid, endi+"l", wdOffset + 124 )
h["LAST_VALID_PNT"] = readX( fid, endi+"l", wdOffset + 128 )
h["FIRST_POINT"] = readX( fid, endi+"l", wdOffset + 132 )
h["SPARSING_FACTOR"] = readX( fid, endi+"l", wdOffset + 136 )
h["SEGMENT_INDEX"] = readX( fid, endi+"l", wdOffset + 140 )
h["SUBARRAY_COUNT"] = readX( fid, endi+"l", wdOffset + 144 )
h["SWEEPS_PER_ACQ"] = readX( fid, endi+"l", wdOffset + 148 )
h["POINTS_PER_PAIR"] = readX( fid, endi+"h", wdOffset + 152 )
h["PAIR_OFFSET"] = readX( fid, endi+"h", wdOffset + 154 )
h["VERTICAL_GAIN"] = readX( fid, endi+"f", wdOffset + 156 ) #to get floating values from raw data :
h["VERTICAL_OFFSET"] = readX( fid, endi+"f", wdOffset + 160 ) #VERTICAL_GAIN * data - VERTICAL_OFFSET
h["MAX_VALUE"] = readX( fid, endi+"f", wdOffset + 164 )
h["MIN_VALUE"] = readX( fid, endi+"f", wdOffset + 168 )
h["NOMINAL_BITS"] = readX( fid, endi+"h", wdOffset + 172 )
h["NOM_SUBARRAY_COUNT"]= readX( fid, endi+"h",wdOffset + 174 )
h["HORIZ_INTERVAL"] = readX( fid, endi+"f", wdOffset + 176 ) #sampling interval for time domain waveforms
h["HORIZ_OFFSET"] = readX( fid, endi+"d", wdOffset + 180 ) #trigger offset for the first sweep of the trigger, seconds between the trigger and the first data point
h["PIXEL_OFFSET"] = readX( fid, endi+"d", wdOffset + 188 )
h["VERTUNIT"] = readX( fid, "48s", wdOffset + 196 ).decode().split('\x00')[0]
h["HORUNIT"] = readX( fid, "48s", wdOffset + 244 ).decode().split('\x00')[0]
h["HORIZ_UNCERTAINTY"]= readX( fid, endi+"f", wdOffset + 292 )
h["TRIGGER_TIME"] = getTimeStamp( fid, endi, wdOffset + 296 )
Year, Month, Day, hour, minute, second = getTimeStampRaw( fid, endi, wdOffset + 296 )
h["TS_Y"] = Year
h["TS_M"] = Month
h["TS_D"] = Day
h["TS_h"] = hour
h["TS_m"] = minute
h["TS_s"] = second
h["ACQ_DURATION"] = readX( fid, endi+"f", wdOffset + 312 )
h["RECORD_TYPE"] = ["single_sweep","interleaved","histogram","graph","filter_coefficient","complex","extrema","sequence_obsolete","centered_RIS","peak_detect"][ readX( fid, endi+"H", wdOffset + 316 ) ]
h["PROCESSING_DONE"] = ["no_processing","fir_filter","interpolated","sparsed","autoscaled","no_result","rolling","cumulative"][ readX( fid, endi+"H", wdOffset + 318 ) ]
h["RIS_SWEEPS"] = readX( fid, endi+"h", wdOffset + 322 )
h["TIMEBASE"] = ['1_ps/div', '2_ps/div', '5_ps/div', '10_ps/div', '20_ps/div', '50_ps/div', '100_ps/div', '200_ps/div', '500_ps/div', '1_ns/div', '2_ns/div', '5_ns/div', '10_ns/div', '20_ns/div', '50_ns/div', '100_ns/div', '200_ns/div', '500_ns/div', '1_us/div', '2_us/div', '5_us/div', '10_us/div', '20_us/div', '50_us/div', '100_us/div', '200_us/div', '500_us/div', '1_ms/div', '2_ms/div', '5_ms/div', '10_ms/div', '20_ms/div', '50_ms/div', '100_ms/div', '200_ms/div', '500_ms/div', '1_s/div', '2_s/div', '5_s/div', '10_s/div', '20_s/div', '50_s/div', '100_s/div', '200_s/div', '500_s/div', '1_ks/div', '2_ks/div', '5_ks/div', 'EXTERNAL'][ readX( fid, endi+"H", wdOffset + 324 ) ]
h["VERT_COUPLING"] = ['DC_50_Ohms', 'ground', 'DC_1MOhm', 'ground', 'AC,_1MOhm'][ readX( fid, endi+"H", wdOffset + 326 ) ]
h["PROBE_ATT"] = readX( fid, endi+"f", wdOffset + 328 )
h["FIXED_VERT_GAIN"] = ['1_uV/div','2_uV/div','5_uV/div','10_uV/div','20_uV/div','50_uV/div','100_uV/div','200_uV/div','500_uV/div','1_mV/div','2_mV/div','5_mV/div','10_mV/div','20_mV/div','50_mV/div','100_mV/div','200_mV/div','500_mV/div','1_V/div','2_V/div','5_V/div','10_V/div','20_V/div','50_V/div','100_V/div','200_V/div','500_V/div','1_kV/div'][ readX( fid, endi+"H", wdOffset + 332 ) ]
h["BANDWIDTH_LIMIT"] = ['off', 'on'][ readX( fid, endi+"H", wdOffset + 334 ) ]
h["VERTICAL_VERNIER"] = readX( fid, endi+"f", wdOffset + 336 )
h["ACQ_VERT_OFFSET"] = readX( fid, endi+"f", wdOffset + 340 )
h["WAVE_SOURCE"] = readX( fid, endi+"H", wdOffset + 344 )
h["USER_TEXT"] = readX( fid, "{0}s".format(lUSER_TEXT), wdOffset + lWAVE_DESCRIPTOR ).decode().split('\x00')[0]
#----------------------------------------------------------
# Get main sample data with the help of numpys .fromfile()
#----------------------------------------------------------
fid.seek( wdOffset + lWAVE_DESCRIPTOR + lUSER_TEXT + lTRIGTIME_ARRAY + lRIS_TIME_ARRAY ) #Seek to WAVE_ARRAY_1
y = np.fromfile( fid, smplFmt, lWAVE_ARRAY_1 )
if endi == ">":
y.byteswap( True )
if raw:
x = np.arange(1,len(y)+1)
else:
y = h["VERTICAL_GAIN"] * y - h["VERTICAL_OFFSET"]
x = np.arange(1,len(y)+1)*h["HORIZ_INTERVAL"] + h["HORIZ_OFFSET"]
return x, y, h, info
def readX( fid, fmt, adr=None ):
""" extract a byte / word / float / double from the binary file """
# struct format definitions
# Format C Type Python type Standard size
# x pad byte no value
# c char string of length 1 1
# b signed char integer 1
# B unsigned char integer 1
# ? _Bool bool 1
# h short integer 2
# H unsigned short integer 2
# i int integer 4
# I unsigned int integer 4
# l long integer 4
# L unsigned long integer 4
# q long long integer 8
# Q unsigned long long integer 8
# f float float 4
# d double float 8
# s char[] string
# p char[] string
# P void * integer
nBytes = struct.calcsize( fmt )
if adr is not None:
fid.seek( adr )
s = struct.unpack( fmt, fid.read( nBytes ) )
if(type(s) == tuple):
return s[0]
else:
return s
def getTimeStamp( fid, endi, adr, debug = None):
""" extract a timestamp from the binary file """
s = readX( fid, endi+"d", adr )
m = readX( fid, endi+"b" )
h = readX( fid, endi+"b" )
D = readX( fid, endi+"b" )
M = readX( fid, endi+"b" )
Y = readX( fid, endi+"h" )
trigTs = datetime.datetime(Y, M, D, h, m, int(s), int((s-int(s))*1e6) )
if debug:
print(" INFO::time", Y, M, D, h, m, s, int(s), int((s-int(s))*1e6))
return trigTs
def getTimeStampRaw( fid, endi, adr, debug = None):
""" extract a timestamp from the binary file """
s = readX( fid, endi+"d", adr )
m = readX( fid, endi+"b" )
h = readX( fid, endi+"b" )
D = readX( fid, endi+"b" )
M = readX( fid, endi+"b" )
Y = readX( fid, endi+"h" )
if debug:
print(" INFO time raw", Y, M, D, h, m, s)
return Y, M, D, h, m, s
<file_sep>import numpy as np
import matplotlib.pyplot as plt
from scipy import stats
#zylinder ohne shaper
#E_real = np.array([80,186,242,295,352,32,662,1173,1332,1063,569])
#E_fit = np.array([0.97844,2.4133,3.137,3.7597,4.3779,0.3457,7.2341,10.7222,11.7675,10.2165,6.485])
#E_real = np.array([569, 662,1173,1332,1063])
#E_fit = np.array([6.472, 7.19,10.703,11.733,10.19])
#FWHM = np.array([0.51, 0.44, 0.87, 0.64, 0.48])
# zylinder mit shaper
E_real = np.array([662,1173,1332,569,1063])
E_fit = np.array([0.753,1.163,1.271,0.7018,1.1080])
FWHM_E = np.array([0.052, 0.069,0.0645, 0.065, 0.055])
#mit shaper ToT
#ToT_real = np.array([662, 1063,1252])
#ToT_fit = np.array([91.00116606316232,95.21278835637344,107.05266288124014])
#FWHM_T = np.array([9.798621093190247,15.77351053281185,22.63164384355587])
#x_err = np.array([0,0,79.5])
#tot mit shaper final
ToT_real = np.array([662,727,1069,1252])
ToT_fit = np.array([77.29,80.00,88.00,91.91])
FWHM_T = np.array([4.26,5,4,11])
#print(ToT_real)
#print(ToT_fit)
#GSI ToT
ToT_GSI = np.array([18.53,18.91,20.73,21.19,22.76])
E_real_GSI = np.array([569,662,1063,1173,1332])
#E_fit_GSI = np.array([0.701803960984309,0.7535521180502402,1.1657715187599953,1.273437535904615])
#ToT_GSI = np.array([18.53,18.95,21.2,22.75])
FWHM_GSI = np.array([1.88,1.68,2.81,3.31,3.46])
#bi207 1069keV 20,2
#plt.plot(E_fit,E_real,ls='None',marker='.')
#plt.errorbar(E_fit,E_real, xerr= 0.5*FWHM_E , fmt='.',capsize = 3,color='k', label='zugeordnete Energie')
#plt.plot(ToT_fit,ToT_real, ls='None', marker='.')
#plt.errorbar(ToT_fit,ToT_real, xerr=0.5*FWHM_T,fmt='.',capsize = 3, label='zugeordnete Energie', color ='k')
#plt.plot(test_tot,test_E, ls='None', marker='.')
plt.errorbar(ToT_GSI,E_real_GSI,xerr=0.5*FWHM_GSI,fmt='.',capsize = 3,color='k', label='zugeordnete Energie')
#Energieauflösung
#E_fit_input = E_fit
#E_real_input = E_real
#FWHM_input = FWHM
#delta_E_fit = np.zeros([len(E_fit_input)])
#delta_E_real = np.zeros(len(E_fit_input))
#for i in range(0, len(E_fit_input), 1):
# print(i)
# delta_E_fit[i] = FWHM_input[i]/E_fit_input[i]
# delta_E_real[i] = delta_E_fit[i]*E_real_input[i]
#plt.plot(E_real, delta_E_fit, ls='None', marker = '.')
#print('%:',delta_E_fit)
#print('absolut:', delta_E_real)
#print(delta_E_fit*100)
from scipy.optimize import curve_fit
def fit_exp(x,a,b):
return a*np.exp(b*x)
def fit_log(x,a,b):
return a*np.log(x)+b
popt, pcov = curve_fit(fit_exp, ToT_GSI , E_real_GSI, bounds=([15,0],[35,1]) ,method ='trf')
#popt,pcov = curve_fit(fit, E_fit, E_real , bounds=([0,0,0],[1000,10,100]), method='trf' )
perr = np.sqrt(np.diag(pcov))
print(popt)
print(perr)
#slope, intercept, r_value, p_value, std_err = stats.linregress(E_fit, E_real)
slope, intercept, r_value, p_value, std_err = stats.linregress(ToT_GSI, E_real_GSI)
fit_data = np.array([slope, intercept])
#print(slope)
#print(intercept)
#print(r_value)
#print(p_value)
#print(std_err)
x_fit_E = np.linspace(17,24,10)
plt.plot(x_fit_E, intercept + slope*x_fit_E, 'r',label='linearer Fit')
x_fit = np.linspace(17,24,1000)
#plt.plot(x_fit, fit_exp(x_fit, *popt), label='exp. Fit',color='r')
plt.legend()
plt.grid()
#plt.text(470,1.2, 'Q(E) = %5.5fE + %5.5f' %tuple(fit_data))
plt.text(17,1200, '$E(T) = m \cdot T + n$')
#plt.text(17,1200, '$E(T)=a \cdot e^{(b \cdot x)}$')
plt.text(17,1125, '$m= 187.69{keV/ns} $')
plt.text(17,1050, '$n= -2873.54 keV $')
plt.title("")
plt.ylabel("Energie E (keV)")
plt.xlabel("ToT T (ns)")
#import tikzplotlib
#tikzplotlib.save("calib_ToT_GSI_linear.tex")
plt.show()
<file_sep>#! /usr/bin/env python3
from __future__ import division
from __future__ import print_function
import os, sys, inspect
cmd_subfolder = os.path.realpath(os.path.abspath(os.path.join(os.path.split(inspect.getfile(inspect.currentframe()))[0],"src")))
if cmd_subfolder not in sys.path:
sys.path.insert(0, cmd_subfolder)
from TLeCroy import * # lecroy.py
import matplotlib.pyplot as plt
from scipy.stats import norm
import numpy as np
import scipy.integrate as integrate
import matplotlib.mlab as mlab
from scipy.signal import find_peaks, peak_widths, peak_prominences
# --------------------------------
# constants to be set by the user
# --------------------------------
channelNr = 4
StartTrace = 0
StopTrace =20
#inName = "--testpulse--"
#inName = "--testpulse--autosave--"
inName = "--testpulse--autosave--segments--"
inDir = "/Users/Lennard/Bachelor_Thesis_LF/BA_Lennard_Franz/cs137-longzylinder/"
#inDir = "/Users/Lennard/Bachelor_Thesis_LF/BA_Lennard_Franz/ra226-longzylinder/"
#inDir = "/volumes/lennad/segment/segment-CeBr-cs137/"
#inDir = "/volumes/lennad/<NAME>/th-zylinder/"
#inDir = "/volumes/lennad/<NAME>/cs137-longzylinder-shaper/"
#inDir = "/Users/Lennard/desktop/osci/lennard/segment-flat-CeBr-CS137/"
#inDir = "/Users/Lennard/desktop/osci/lennard/cs137-longzylinder-shaper/"
#inDir = "/Users/Lennard/destop/osci/lennard/cebr-big-4ch-cs137/"
#inDir = "/Users/Lennard/desktop/osci/lennard/segment-CeBr-ra226/"
#inDir = "/Users/Lennard/desktop/osci/lennard/ra226-filter/"
#inDir = "/Users/Lennard/desktop/autosave-segments/"
#inDir = "/Users/Lennard/desktop/osci/lennard/gag-am241/"
#inDir = "/home/lfranz/work/osci/lennard/segment-longcable-CeBr-CS137/"
#inDir = "/home/lfranz/work/osci/lennard/segment-flat-CeBr-CS137/"
#inDir = "/home/lfranz/work/osci/lennard/segment-flat-plexi-background/"
#inDir = "/home/lfranz/work/osci/lennard/segment-CeBr-am241/"
#inDir = "/home/lfranz/work/osci/lennard/segment-CeBr-cs137/"
#inDir = "/home/lfranz/work/osci/lennard/segment-CeBr-ra226/"
#inDir = "/home/lfranz/work/osci/lennard/segment/"
#inDir = "/home/lfranz/work/autosave-segments/"
#inDir = "/ZIH.fast/projects/d-lab_data/MAPMT/2019_gsi/segment/"
#inDir = "/ZIH.fast/projects/d-lab_data/MAPMT/2019_gsi/segments-new/"
#inDir = "/ZIH.fast/projects/d-lab_data/MAPMT/2019_gsi/segments-new/Autosave-new/"
# inDir = "/ZIH.fast/projects/d-lab_data/MAPMT/2019_gsi/Autosave/"
# --------------------------------
import numpy as np
import scipy.integrate as integrate
#empty array for area of sequnces for every trace
A = np.empty([0])
print(inDir)
for traceNr in range(StartTrace, StopTrace + 1, 1):
print(" INFO: working on trace: ", "C"+ str(channelNr).zfill(1) + inName + str(traceNr).zfill(5) + ".trc")
fileName = inDir + "C" + str(channelNr).zfill(1) + inName + str(traceNr).zfill(5) + ".trc"
TLC = TLeCroy(fileName, debug=True)
#TLC.PrintPrivate()
# TLC.PlotTrace(raw=False)
header = TLC.GetHeader()
x, y = TLC.GetTrace()
y = y - header['VERTICAL_OFFSET']
y = -y
nano = 1e-9
#print('----------------')
#print(' vertical gain:', header['VERTICAL_GAIN'])
#print('----------------')
seqLength = header['WAVE_ARRAY_COUNT'] // header['SUBARRAY_COUNT']
seqFirst = header['FIRST_VALID_PNT']
#print(' LENGTH', seqLength)
#plt.plot(x / nano, y)
#arrays
idxHigh = np.zeros(header['SUBARRAY_COUNT'],dtype=np.int)
idxLow = np.zeros(header['SUBARRAY_COUNT'],dtype=np.int)
mean = np.zeros(header['SUBARRAY_COUNT'])
std = np.zeros(header['SUBARRAY_COUNT'])
area = np.zeros(header['SUBARRAY_COUNT'])
area_all = np.zeros(header['SUBARRAY_COUNT'])
area_all_sum = np.zeros(header['SUBARRAY_COUNT'])
area_bool =np.zeros(header['SUBARRAY_COUNT'])
tot = np.zeros(header['SUBARRAY_COUNT'])
# determine background
for seqNr in range(1, header['SUBARRAY_COUNT'] + 1):
#bondarys of sequences
idxHigh[seqNr-1] = seqFirst +( seqLength * seqNr - 1)
idxLow[seqNr-1] = idxHigh[seqNr-1] - seqLength + 1
#finding basline
#y[...] has to be adjusted depending vertical position of the puls
mean[seqNr-1], std[seqNr-1] = norm.fit(y[ idxLow[seqNr-1] : idxLow[seqNr-1] + int(0.35*seqLength)])
mean_all = sum(mean)/header['SUBARRAY_COUNT']
std_all = sum(std)/header['SUBARRAY_COUNT']
#Histogram of values for baseline
#plt.hist(y[ idxLow[seqNr-1] : idxLow[seqNr-1] + int(0.35*seqLength)])
#determination of area of pulses
for seqNr in range(1, header['SUBARRAY_COUNT'] + 1):
# if mean[seqNr -1] > 0.0285 and mean[seqNr - 1] < 0.0315 and np.min( y[idxLow[seqNr-1] : idxHigh[seqNr-1]]-mean[seqNr-1]) > -0.0035:
#area of the pulse
#area[seqNr-1] = np.trapz((y[idxLow[seqNr - 1]:idxHigh[seqNr - 1]]- mean[seqNr-1]) > 3*std[seqNr-1] ,
#x[idxLow[seqNr - 1]:idxHigh[seqNr - 1]]/nano)
#area_all[seqNr-1] = np.sum(y[idxLow[seqNr - 1] : idxHigh[seqNr - 1]])/(nano/header['HORIZ_INTERVAL']) - ((mean_all)*seqLength*header['HORIZ_INTERVAL']/nano)
#print(area_all_sum)
#print(area_all)
#condition to differentiate values of pulse from background
#boolArr = np.where((y[idxLow[seqNr - 1]:idxHigh[seqNr - 1]]- mean[seqNr - 1]) > (3*std[seqNr -1]))
boolArr = (y[idxLow[seqNr - 1]:idxHigh[seqNr - 1]]- mean[seqNr-1]) > (3*std[seqNr-1])
#sum of all values which meet condition and scaling
#substracting of area belonging to background
#print(y[boolArr[0]] - mean[seqNr-1])
#area_bool[seqNr-1] = np.sum(y[boolArr[0]])*(header['HORIZ_INTERVAL']/nano) - (mean[seqNr-1])*(len(boolArr[0])*header['HORIZ_INTERVAL']/nano)
area_bool[seqNr-1] = np.sum((y[idxLow[seqNr - 1]:idxHigh[seqNr - 1]]*(header['HORIZ_INTERVAL']/nano)),where = boolArr) - mean[seqNr-1]*np.sum(boolArr)*header['HORIZ_INTERVAL']/nano
tot[seqNr-1] = (np.sum(boolArr)-1)*header['HORIZ_INTERVAL']/nano
#print(area_bool[seqNr - 1],tot[seqNr - 1] )
#area_bool[seqNr-1] = np.sum(y[boolArr[0]])
#- (mean[seqNr-1])*(len(boolArr[0]))
#print(len(boolArr[0])*header['HORIZ_INTERVAL']/nano)
#plt.hist(len(boolArr[0])*header['HORIZ_INTERVAL']/nano)
#plt.plot(x[idxLow[seqNr - 1]:idxHigh[seqNr - 1]] / nano, y[idxLow[seqNr - 1]:idxHigh[seqNr - 1]]- mean_all)
# plt.plot(x[idxLow[2]:idxHigh[2]] / nano, y[idxLow[2]:idxHigh[2]]- mean_all)
#print("-----------------------")
#print("mean all:",mean_all,"sigma all:", std_all)
#print("array of mean of segments:",mean,"array of sigma of segments",std)
#print("-----------------------")
#appending area of segments to A for every trace
#length of A is number of sequnces * number of traces
#print(y[boolArr[0]] - mean[seqNr-1])
A = np.append(A,area_bool)
#<NAME>
E_real = np.array([569, 662,1173,1332,1063])
E_fit = np.array([6.485, 7.2341,10.7222,11.7675,10.2165])
#E_real = np.array([80,186,242,295,352,32,662,1173,1332,1063,569])
#E_fit = np.array([0.97844,2.4133,3.137,3.7597,4.3779,0.3457,7.2341,10.7222,11.7675,10.2165,6.485])
#mit shaper
#E_real = np.array([662,1173,1332,569,1063])
#E_fit = np.array([0.7535521180502402,1.1657715187599953,1.273437535904615,0.701803960984309,1.1086378999695787])
from scipy import stats
slope, intercept, r_value, p_value, std_err = stats.linregress(E_fit, E_real)
#shaper wolfram alpha
#slope = 1298.14
#intercept = -339.482
#shaper python
#slope = 1298.93696929096
#intercept= -340.41065484554497
#no shaper wolfram
#slope = 143.049
#intercept = -363.694
print(intercept)
print(slope)
E = intercept+slope*A
print(E)
#histogram of all sequences of all traces
n,bins,patches = plt.hist(E,bins=500,histtype='step', color='k')
#print(n,bins,patches)
plt.grid(True)
bin_width= (bins[1]-bins[0])
#plt.xlim(0.,15.0)
peaks,properties = find_peaks(n,width=3, distance=1,height=300, rel_height=0.7)
print("bins of peaks:", peaks)
print("X Values of peaks:", bins[peaks] )
print(properties)
#print(bins[peaks])
#print(bins[np.int(peaks[6] - 1.5* properties["widths"][6]) :np.int( peaks[6]+ 1.5* (properties["widths"][6])) ])
#print(peaks[2] - 1.5* properties["widths"][2])
#print(np.int(peaks[2] - 1.5* properties["widths"][2]))
#print(n,bins)
#peakNr = 6
#print (bins[np.int(peaks[peakNr] - 1.5 * properties["widths"][peakNr]) :np.int( peaks[peakNr]+ 1.5 * (properties["widths"][peakNr])) ])
#print (n[np.int(peaks[peakNr] - 1.5* properties["widths"][peakNr]) :np.int( peaks[peakNr]+ 1.5* (properties["widths"][peakNr])) ] )
from scipy.optimize import curve_fit
def gauss (x,a,sigma,mu):
#return (1/np.sqrt(2*np.pi*sigma**2))* np.exp(- (x-mu)**2 / 2*sigma**2)
return a*np.exp(-(x-mu)**2/(2*sigma**2))
bins = np.delete(bins,[len(bins)-1])
for peakNr in range(1, len(peaks) ):
fit_width = 0.7
x_values = bins[np.int(peaks[peakNr] - fit_width * properties["widths"][peakNr]) :np.int( peaks[peakNr]+ fit_width * (properties["widths"][peakNr])) ]
y_values = n[np.int(peaks[peakNr] - fit_width * properties["widths"][peakNr]) :np.int( peaks[peakNr]+ fit_width * (properties["widths"][peakNr])) ]
#print(x_values)
#print(y_values)
a_bound = [0, 1.1*np.max(y_values)]
sigma_bound = [0, 50]
mu_bound = [np.min(x_values), np.max(x_values)]
#print(a_bound)
#print(sigma_bound)
#print(mu_bound)
#popt, pcov = curve_fit(gauss, bins[np.int(peaks[peakNr] - 1.5 * properties["widths"][peakNr]) :np.int( peaks[peakNr]+ 1.5 * (properties["widths"][peakNr])) ] , n[np.int(peaks[peakNr] - 1.5* properties["widths"][peakNr]) :np.int( peaks[peakNr]+ 1.5* (properties["widths"][peakNr])) ] )
popt, pcov = curve_fit(gauss, x_values , y_values, bounds= ([a_bound[0],sigma_bound[0],mu_bound[0]], [a_bound[1],sigma_bound[1],mu_bound[1]] ) )
plt.plot(bins, gauss(bins, *popt),color='g')
plt.plot((popt[2], popt[2]), (0, 1600), 'r-')
#statistic error of curve fit
perr = np.sqrt(np.diag(pcov))
#FWHM
FWHM = 2* np.sqrt(2*np.log(2)) * popt[1]
delta_FWHM = 2* np.sqrt(2*np.log(2)) * perr[1]
print("Peak Nr:" , peakNr)
print("Energy:" , popt[2], "statistic error of energy:" , perr[2], "FWHM:", FWHM, "statistic error of FWHM", delta_FWHM )
plt.title("Histogramm der deponierten Ladung im Detektor")
plt.xlabel("deponierte Ladung (nVs)")
plt.ylabel("Anzahl N ")
plt.xlim(-500,1000)
plt.ylim(0,1300)
#determination of mean and sigma of data in histogram
#MEAN, STD = norm.fit(A)
#print("--------------------------")
#print("Entries:" , len(A))
#print("--------------------------")
#print("Mean of pulse energy :", MEAN ,"Sigma of pulse energy:", STD)
#print("--------------------------")
plt.show()
<file_sep>#! /usr/bin/env python3
from __future__ import division
from __future__ import print_function
import os, sys, inspect
cmd_subfolder = os.path.realpath(os.path.abspath(os.path.join(os.path.split(inspect.getfile(inspect.currentframe()))[0],"src")))
if cmd_subfolder not in sys.path:
sys.path.insert(0, cmd_subfolder)
from TLeCroy import * # lecroy.py
import matplotlib.pyplot as plt
from scipy.stats import norm
import numpy as np
import scipy.integrate as integrate
import matplotlib.mlab as mlab
from scipy.signal import find_peaks
# --------------------------------
# constants to be set by the user
# --------------------------------
channelNr = 4
StartTrace = 0
StopTrace = 25
#inName = "--testpulse--"
#inName = "--testpulse--autosave--"
inName = "--testpulse--autosave--segments--"
inDir = "/Users/Lennard/Bachelor_Thesis_LF/BA_Lennard_Franz/cs137-longzylinder/"
#inDir = "/volumes/lennad/segment/segment-CeBr-cs137/"
#inDir = "/volumes/lennad/<NAME>anz/cs137-longzylinder/"
#inDir = "/volumes/lennad/<NAME>anz/th232-cebr-4x4/"
#inDir = "/Users/Lennard/desktop/osci/lennard/segment-flat-CeBr-CS137/"
#inDir = "/Users/Lennard/desktop/osci/lennard/cs137-longzylinder-shaper/"
#inDir = "/Users/Lennard/destop/osci/lennard/cebr-big-4ch-cs137/"
#inDir = "/Users/Lennard/desktop/osci/lennard/segment-CeBr-am241/"
#inDir = "/Users/Lennard/desktop/osci/lennard/ra226-filter/"
#inDir = "/Users/Lennard/desktop/autosave-segments/"
#inDir = "/Users/Lennard/desktop/osci/lennard/gag-am241/"
#inDir = "/home/lfranz/work/osci/lennard/segment-longcable-CeBr-CS137/"
#inDir = "/home/lfranz/work/osci/lennard/segment-flat-CeBr-CS137/"
#inDir = "/home/lfranz/work/osci/lennard/segment-flat-plexi-background/"
#inDir = "/home/lfranz/work/osci/lennard/segment-CeBr-am241/"
#inDir = "/home/lfranz/work/osci/lennard/segment-CeBr-cs137/"
#inDir = "/home/lfranz/work/osci/lennard/segment-CeBr-ra226/"
#inDir = "/home/lfranz/work/osci/lennard/segment/"
#inDir = "/home/lfranz/work/autosave-segments/"
#inDir = "/ZIH.fast/projects/d-lab_data/MAPMT/2019_gsi/segment/"
#inDir = "/ZIH.fast/projects/d-lab_data/MAPMT/2019_gsi/segments-new/"
#inDir = "/ZIH.fast/projects/d-lab_data/MAPMT/2019_gsi/segments-new/Autosave-new/"
# inDir = "/ZIH.fast/projects/d-lab_data/MAPMT/2019_gsi/Autosave/"
# --------------------------------
import numpy as np
import scipy.integrate as integrate
#empty array for area of sequnces for every trace
A = np.empty([0])
print(inDir)
for traceNr in range(StartTrace, StopTrace + 1, 1):
print(" INFO: working on trace: ", "C"+ str(channelNr).zfill(1) + inName + str(traceNr).zfill(5) + ".trc")
fileName = inDir + "C" + str(channelNr).zfill(1) + inName + str(traceNr).zfill(5) + ".trc"
TLC = TLeCroy(fileName, debug=True)
#TLC.PrintPrivate()
# TLC.PlotTrace(raw=False)
header = TLC.GetHeader()
x, y = TLC.GetTrace()
y = y - header['VERTICAL_OFFSET']
y = -y
nano = 1e-9
#print('----------------')
#print(' vertical gain:', header['VERTICAL_GAIN'])
#print('----------------')
seqLength = header['WAVE_ARRAY_COUNT'] // header['SUBARRAY_COUNT']
seqFirst = header['FIRST_VALID_PNT']
#print(' LENGTH', seqLength)
#plt.plot(x / nano, y)
#arrays
idxHigh = np.zeros(header['SUBARRAY_COUNT'],dtype=np.int)
idxLow = np.zeros(header['SUBARRAY_COUNT'],dtype=np.int)
mean = np.zeros(header['SUBARRAY_COUNT'])
std = np.zeros(header['SUBARRAY_COUNT'])
area = np.zeros(header['SUBARRAY_COUNT'])
area_all = np.zeros(header['SUBARRAY_COUNT'])
area_all_sum = np.zeros(header['SUBARRAY_COUNT'])
area_bool =np.zeros(header['SUBARRAY_COUNT'])
# determine background
for seqNr in range(1, header['SUBARRAY_COUNT'] + 1):
#bondarys of sequences
idxHigh[seqNr-1] = seqFirst +( seqLength * seqNr - 1)
idxLow[seqNr-1] = idxHigh[seqNr-1] - seqLength + 1
#finding basline
#y[...] has to be adjusted depending vertical position of the puls
mean[seqNr-1], std[seqNr-1] = norm.fit(y[ idxLow[seqNr-1] : idxLow[seqNr-1] + int(0.35*seqLength)])
mean_all = sum(mean)/header['SUBARRAY_COUNT']
std_all = sum(std)/header['SUBARRAY_COUNT']
#Histogram of values for baseline
#plt.hist(y[ idxLow[seqNr-1] : idxLow[seqNr-1] + int(0.35*seqLength)])
#determination of area of pulses
for seqNr in range(1, header['SUBARRAY_COUNT'] + 1):
# if mean[seqNr -1] > 0.0285 and mean[seqNr - 1] < 0.0315 and np.min( y[idxLow[seqNr-1] : idxHigh[seqNr-1]]-mean[seqNr-1]) > -0.0035:
#area of the pulse
#area[seqNr-1] = np.trapz((y[idxLow[seqNr - 1]:idxHigh[seqNr - 1]]- mean[seqNr-1]) > 3*std[seqNr-1] ,
#x[idxLow[seqNr - 1]:idxHigh[seqNr - 1]]/nano)
#area_all[seqNr-1] = np.sum(y[idxLow[seqNr - 1] : idxHigh[seqNr - 1]])/(nano/header['HORIZ_INTERVAL']) - ((mean_all)*seqLength*header['HORIZ_INTERVAL']/nano)
#print(area_all_sum)
#print(area_all)
#condition to differentiate values of pulse from background
#boolArr = np.where((y[idxLow[seqNr - 1]:idxHigh[seqNr - 1]]- mean[seqNr - 1]) > (3*std[seqNr -1]))
boolArr = (y[idxLow[seqNr - 1]:idxHigh[seqNr - 1]]- mean[seqNr-1]) > (3*std[seqNr-1])
#sum of all values which meet condition and scaling
#substracting of area belonging to background
#print(y[boolArr[0]] - mean[seqNr-1])
#area_bool[seqNr-1] = np.sum(y[boolArr[0]])*(header['HORIZ_INTERVAL']/nano) - (mean[seqNr-1])*(len(boolArr[0])*header['HORIZ_INTERVAL']/nano)
area_bool[seqNr-1] = np.sum((y[idxLow[seqNr - 1]:idxHigh[seqNr - 1]]*(header['HORIZ_INTERVAL']/nano)),where = boolArr) - mean[seqNr-1]*np.sum(boolArr)*header['HORIZ_INTERVAL']/nano
#area_bool[seqNr-1] = np.sum(y[boolArr[0]])
#- (mean[seqNr-1])*(len(boolArr[0]))
#print(len(boolArr[0])*header['HORIZ_INTERVAL']/nano)
#plt.hist(len(boolArr[0])*header['HORIZ_INTERVAL']/nano)
#plt.plot(x[idxLow[seqNr - 1]:idxHigh[seqNr - 1]] / nano, y[idxLow[seqNr - 1]:idxHigh[seqNr - 1]]- mean_all)
# plt.plot(x[idxLow[2]:idxHigh[2]] / nano, y[idxLow[2]:idxHigh[2]]- mean_all)
#print("-----------------------")
#print("mean all:",mean_all,"sigma all:", std_all)
#print("array of mean of segments:",mean,"array of sigma of segments",std)
#print("-----------------------")
#appending area of segments to A for every trace
#length of A is number of sequnces * number of traces
#print(y[boolArr[0]] - mean[seqNr-1])
A = np.append(A,area_bool)
#peaks = find_peaks_cwt(A)
#print(peaks)
#histogram of all sequences of all traces
n,bins,patches=plt.hist(A,bins=1000,histtype='step', color = 'k')
#print(n,bins)
plt.grid(True)
plt.xlim(0.,10.0)
peaks = find_peaks(n,height=200, width=2, distance=5)
print( bins[peaks[0]])
plt.title("histogram of pulse energy")
plt.xlabel("energy [nVs]")
plt.ylabel("counts N")
#determination of mean and sigma of data in histogram
MEAN, STD = norm.fit(A)
#print("--------------------------")
#print("Entries:" , len(A))
#print("--------------------------")
#print("Mean of pulse energy :", MEAN ,"Sigma of pulse energy:", STD)
#print("--------------------------")
plt.show()
| f73503937eb61fd1a4ebf1211e48ebaf0164418a | [
"Python"
] | 8 | Python | LennardFranz/BT_LF | 4f80a3a18a6f49a663a5dab833c8293fc459601c | d38881b41cc8fdf05f3ffcd97d491b4ea405412c | |
refs/heads/master | <file_sep>import logo from './logo.svg';
import s from './App.module.scss';
import card from './news_foto.jpg'
function App() {
return (
<div className={s.App}>
<div className={s.card}>
<h3 className={s.cardLabel}>Семантика в верстке</h3>
<div className={s.content}>
<div className={s.img}><img src={card} alt=""/></div>
<div className={s.info}>
<h6 className={s.infoLabel}><a href="">htmlbook</a> - <span>5 января 2014</span></h6>
<p>Семантика дает представление о структуре документа и позволяет людям и программам более полно управлять данными. В HTML5 добавлено множество семантических тегов, а также поддержка RDFa, микроформаторов и микроданных ...</p>
</div>
<a href="" className={s.readMoreLink}>ЧИТАТЬ ДАЛЬШЕ</a>
</div>
</div>
<div className={s.card}>
<h3 className={s.cardLabel}>Семантика в верстке</h3>
<div className={s.content}>
<div className={s.img}><img src={card} alt=""/></div>
<div className={s.info}>
<h6 className={s.infoLabel}><a href="">htmlbook</a> - <span>5 января 2014</span></h6>
<p>Семантика дает представление о структуре документа и позволяет людям и программам более полно управлять данными. В HTML5 добавлено множество семантических тегов, а также поддержка RDFa, микроформаторов и микроданных ...</p>
</div>
<a href="" className={s.readMoreLink}>ЧИТАТЬ ДАЛЬШЕ</a>
</div>
</div>
<div className={s.card}>
<h3 className={s.cardLabel}>Семантика в верстке</h3>
<div className={s.content}>
<div className={s.img}><img src={card} alt=""/></div>
<div className={s.info}>
<h6 className={s.infoLabel}><a href="">htmlbook</a> - <span>5 января 2014</span></h6>
<p>Семантика дает представление о структуре документа и позволяет людям и программам более полно управлять данными. В HTML5 добавлено множество семантических тегов, а также поддержка RDFa, микроформаторов и микроданных ...</p>
</div>
<a href="" className={s.readMoreLink}>ЧИТАТЬ ДАЛЬШЕ</a>
</div>
</div>
<div className={s.card}>
<h3 className={s.cardLabel}>Семантика в верстке</h3>
<div className={s.content}>
<div className={s.img}><img src={card} alt=""/></div>
<div className={s.info}>
<h6 className={s.infoLabel}><a href="">htmlbook</a> - <span>5 января 2014</span></h6>
<p>Семантика дает представление о структуре документа и позволяет людям и программам более полно управлять данными. В HTML5 добавлено множество семантических тегов, а также поддержка RDFa, микроформаторов и микроданных ...</p>
</div>
<a href="" className={s.readMoreLink}>ЧИТАТЬ ДАЛЬШЕ</a>
</div>
</div>
<div className={s.card}>
<h3 className={s.cardLabel}>Семантика в верстке</h3>
<div className={s.content}>
<div className={s.img}><img src={card} alt=""/></div>
<div className={s.info}>
<h6 className={s.infoLabel}><a href="">htmlbook</a> - <span>5 января 2014</span></h6>
<p>Семантика дает представление о структуре документа и позволяет людям и программам более полно управлять данными. В HTML5 добавлено множество семантических тегов, а также поддержка RDFa, микроформаторов и микроданных ...</p>
</div>
<a href="" className={s.readMoreLink}>ЧИТАТЬ ДАЛЬШЕ</a>
</div>
</div>
</div>
);
}
export default App;
| 0a3b80b7ae34e52a141551c2f1828d524daa52a9 | [
"JavaScript"
] | 1 | JavaScript | sinvorgena/TestTaskForColibri | 55332d0eb9127b88259710d5b3d8c205836aa6e6 | 9140513762f677f8bafebb3e1f8af2d365da001f |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.