branch_name
stringclasses 15
values | target
stringlengths 26
10.3M
| directory_id
stringlengths 40
40
| languages
sequencelengths 1
9
| num_files
int64 1
1.47k
| repo_language
stringclasses 34
values | repo_name
stringlengths 6
91
| revision_id
stringlengths 40
40
| snapshot_id
stringlengths 40
40
| input
stringclasses 1
value |
---|---|---|---|---|---|---|---|---|---|
refs/heads/master | <file_sep>////////////////////////////////////////////////////////////////////////////////
//
// *** Helper code to convert from UTF-8 to UTF-16 ***
//
// by <NAME>
//
//
// This code is based on my MSDN Magazine article published
// on the 2016 September issue:
//
// "C++ - Unicode Encoding Conversions with STL Strings and Win32 APIs"
// https://msdn.microsoft.com/magazine/mt763237
//
////////////////////////////////////////////////////////////////////////////////
#pragma once
#include <limits> // std::numeric_limits
#include <stdexcept> // std::runtime_error, std::overflow_error
#include <string> // std::string, std::wstring
#include <crtdbg.h> // _ASSERTE()
#include <Windows.h> // Win32 Platform SDK main header
//------------------------------------------------------------------------------
// Error when converting from UTF-8 to UTF-16
//------------------------------------------------------------------------------
class Utf16FromUtf8ConversionError
: public std::runtime_error
{
public:
// Initialize with error message raw C-string and last Win32 error code
Utf16FromUtf8ConversionError(const char* message, DWORD errorCode)
: std::runtime_error(message)
, m_errorCode(errorCode)
{}
// Initialize with error message string and last Win32 error code
Utf16FromUtf8ConversionError(const std::string& message, DWORD errorCode)
: std::runtime_error(message)
, m_errorCode(errorCode)
{}
// Retrieve error code associated to the failed conversion
DWORD ErrorCode() const
{
return m_errorCode;
}
private:
// Error code from GetLastError()
DWORD m_errorCode;
};
//------------------------------------------------------------------------------
// Convert from UTF-8 to UTF-16.
// Throws Utf16FromUtf8ConversionError on error.
//------------------------------------------------------------------------------
inline std::wstring Utf16FromUtf8(const std::string& utf8)
{
// Result of the conversion
std::wstring utf16;
// First, handle the special case of empty input string
if (utf8.empty())
{
_ASSERTE(utf16.empty());
return utf16;
}
// Safely fail if an invalid UTF-8 character sequence is encountered
const DWORD kFlags = MB_ERR_INVALID_CHARS;
// Safely cast the length of the source UTF-8 string (expressed in chars)
// from size_t (returned by std::string::length()) to int
// for the MultiByteToWideChar API.
// If the size_t value is too big to be stored into an int,
// throw an exception to prevent conversion errors like huge size_t values
// converted to *negative* integers.
if (utf8.length() > static_cast<size_t>((std::numeric_limits<int>::max)()))
{
throw std::overflow_error(
"Input string too long: size_t-length doesn't fit into int.");
}
const int utf8Length = static_cast<int>(utf8.length());
// Get the size of the destination UTF-16 string
const int utf16Length = ::MultiByteToWideChar(
CP_UTF8, // source string is in UTF-8
kFlags, // conversion flags
utf8.data(), // source UTF-8 string pointer
utf8Length, // length of the source UTF-8 string, in chars
nullptr, // unused - no conversion done in this step
0 // request size of destination buffer, in wchar_ts
);
if (utf16Length == 0)
{
// Conversion error: capture error code and throw
const DWORD error = ::GetLastError();
throw Utf16FromUtf8ConversionError(
error == ERROR_NO_UNICODE_TRANSLATION ?
"Invalid UTF-8 sequence found in input string."
:
"Cannot get result string length when converting " \
"from UTF-8 to UTF-16 (MultiByteToWideChar failed).",
error
);
}
// Make room in the destination string for the converted bits
utf16.resize(utf16Length);
// Do the actual conversion from UTF-8 to UTF-16
int result = ::MultiByteToWideChar(
CP_UTF8, // source string is in UTF-8
kFlags, // conversion flags
utf8.data(), // source UTF-8 string pointer
utf8Length, // length of source UTF-8 string, in chars
&utf16[0], // pointer to destination buffer
utf16Length // size of destination buffer, in wchar_ts
);
if (result == 0)
{
// Conversion error: capture error code and throw
const DWORD error = ::GetLastError();
throw Utf16FromUtf8ConversionError(
error == ERROR_NO_UNICODE_TRANSLATION ?
"Invalid UTF-8 sequence found in input string."
:
"Cannot convert from UTF-8 to UTF-16 "\
"(MultiByteToWideChar failed).",
error
);
}
return utf16;
}
////////////////////////////////////////////////////////////////////////////////
<file_sep>////////////////////////////////////////////////////////////////////////////////
//
// Test printing UTF-8-encoded text to the Windows console
//
// by <NAME>
//
////////////////////////////////////////////////////////////////////////////////
#include "UnicodeConv.hpp" // My Unicode conversion helpers
#include <fcntl.h>
#include <io.h>
#include <stdint.h>
#include <iostream>
#include <string>
int main()
{
// Change stdout to Unicode UTF-16.
//
// Note: _O_U8TEXT doesn't seem to work, e.g.:
// https://blogs.msmvps.com/gdicanio/2017/08/22/printing-utf-8-text-to-the-windows-console/
//
_setmode(_fileno(stdout), _O_U16TEXT);
// Japanese name for Japan, encoded in UTF-8
uint8_t utf8[] = {
0xE6, 0x97, 0xA5, // U+65E5
0xE6, 0x9C, 0xAC, // U+672C
0x00
};
std::string japan(reinterpret_cast<const char*>(utf8));
// Print UTF-16-encoded text
std::wcout << Utf16FromUtf8("Japan") << L"\n\n";
std::wcout << Utf16FromUtf8(japan) << L'\n';
}
////////////////////////////////////////////////////////////////////////////////
<file_sep># PrintUtf8ToWindowsConsole
Demo code printing UTF-8-encoded text to the Windows console
by <NAME>
This repo is associated to this blog post:
https://blogs.msmvps.com/gdicanio/2019/04/09/printing-utf-8-text-to-the-windows-console-sample-code/
| 6e43477b5fc2d61a2bcc2d1ebc7366617fce13a4 | [
"Markdown",
"C++"
] | 3 | C++ | GiovanniDicanio/PrintUtf8ToWindowsConsole | 134bcef548cf0a55d155e56ced52863540f48f06 | aacf7b6c56573472d0db4de98df2b1913ae3b84c | |
refs/heads/main | <file_sep># An array in programming is an indexed collection of related items
# Lets put some capitals of countries in an array
capitals = ["London", "Paris", "Berlin"]
# You can access any element in an array by specifying its index.
capitals[2]
# Will give you "Berlin"
capitals[0]
# Will give you "London"
capitals[-1]
# Will give you "Berlin"
# You can also use first or last method on arrays
capitals.first
# Will give you "London"
capitals.last
# Will give you "Berlin"
# Commonly user methods on arrays
# pop
# pop will remove the last element on an array and return the array if nothing is specified
capitals.pop
# Will give you ["London","Paris"]
capitals.pop(2)
# This will give you the last two elements in an array into a new array
# to_h
# This command will return a new hash formed from self
a = [3,4,5,6, [2,4,5], :sym]
b = a.to_h
puts b
# each
# iterates over each element in an array
a = [:foo, 'bar', 2]
a.each { |element| puts "#{element.class} #{element}" }
# the output from using this will be
# Symbol foo
# String bar
# Integer 2
<file_sep># The next segment im now going to take a look at some of the methods used in hashes and then move on from there
# key and keys
# key returns the specific key on what position it is in the hash
h = {foo: 0, bar: 2, baz: 2}
h.key(0) # => :foo
h.key(2) # => :bar
# keys returns a new array containing all the keys in self
h.keys # => [:foo, :bar, :baz]
# invert
# invert returns a new hash object with the key and value pair inverted
h1 = h.invert # => {0 => :foo, 1=> :bar, 2 => baz:}
| 59552d7215c839a7e5c0fb8fddd20200dc5317e4 | [
"Ruby"
] | 2 | Ruby | LCzoboriek/Makers-Pills | 583758ac87aba4e64a09e6c5d2884642d679c6aa | 3d7dea765e4065db9070b566cca24cca7c1866f4 | |
refs/heads/master | <file_sep>/*
Find Max Subarray by Enumeration
Loop over each pair of indices
i, j and compute the sum,
Sigma(f)(k=i) a[k]
Keep the best sum you have found so far
*/
#include <stdio.h>
/*
Pre: a[] is an array of integers of length n
Post: a[] is now the max subarray
Returns: int which represents greatest sum in
subarray
*/
int enumMaxSubarray(int a[], int n){
//counters
int i; //pos of first term in subarray
int j; //last term in subarray
int k; //current term in subarray
//int currentArray [n];
int currentArrayLength;
//int maxArray [n];
int maxArrayLength;
int sum = 0;
int best = 0;
for (i = 0; i < n; i++) //first term
{
for (j = i; j < n; j++){//last term
sum = 0;//
//eraseArray(currentArray);
currentArrayLength = 0;
for(k = i; k <= j; k++) //step thru terms
{
sum += a[k];
//currentArray[(k-i)] = a[k];
currentArrayLength++;
}
if (sum > best){
best = sum; //new record
maxArrayLength = currentArrayLength;
//copyArray(currentrrentArray, maxArray, maxArrayLength);
}
}
}
return best;
}
<file_sep>
TODO
Algorithms
- enumeration
- [x] enumeration (a1.c) returns correct sum
- [x] a1.c returns correct sum for test file
- better enum
- [x] a2.c returns correct sum
- [x] a2.c tested with test file
- div and conq
- [x] a3.c seems to return correct sum
- [x] a3.c tested with test file
Analysis
- [x] a1.c analysis data collected
- [x] a2.c analysis data collected
- [x] a3.c analysis data collected
Report
- Mathematical analysis
-
- pseudocode
- [x] a1 pseudocode
- [x] a2 pseudocode
- [x] a3 pseudocode
- [x] asymptomatic run times
- Theoretical correctness
- [ ] a3 proof
- Testing
- [x] results of testing against document
- Experimental analysis
- [x] plots
- [ ] what else?
- Extrapolation and interpretation
- [x] biggest size arrays solvable in an hour
- [ ] experimental results vs theoretical
<file_sep>/*
Finding the maximum sub-array
Divide and Conquer
If we split the array into two halves, we know that the maximum
subarray will either be
• contained entirely i n the first half,
•contained entirely in the second half, or
•made of a suffix of the first half of maximum sum and a prefix of the second half of maximum sum
The first two cases can be found recursively. The last case can be found in linear time
*/
#include <stdio.h>
#include <stdlib.h>
#include <math.h> //fmax
int divAndConMaxSubarray(int a[], int lo, int hi)
{
//int* sumArray = malloc((hi - lo) * sizeof(int)); //for storing winning array,
//maybe don't need
//midpoint of array
int midpoint;
//max's found w/ div and conq
int leftMax=0;
int rightMax=0;
//max's found crossing middle iteratively
int bothMax=0;
int bothMaxLeft=0;
int bothMaxRight=0;
//counters
int i, sum;
//base case, only 1 element in array
//TODO: ensure no issue w/ no elements
if (lo==hi) { //lo and hi are the same element
return a[hi]; //sum is this element alone
}
//divide array into two halves
midpoint = (lo + hi)/2;
//maximum of
//maximum sum on left
//recursive call
leftMax = divAndConMaxSubarray(a, lo, midpoint);
//max sum on right
//recursive call
rightMax = divAndConMaxSubarray(a, midpoint+1, hi);
//max sum from midpoint
//find max going left
bothMaxLeft = 0;
sum = 0;
for (i = midpoint-1; i >=0; i-- ){
sum += a[i];
if (sum > bothMaxLeft)
bothMaxLeft = sum;
}
//find max going right
bothMaxRight = 0;
sum = 0;
for (i = midpoint; i < hi; i++ ){
sum += a[i];
if (sum > bothMaxRight)
bothMaxRight = sum;
}
//combine
bothMax = bothMaxRight + bothMaxLeft;
return fmax(bothMax, fmax(leftMax, rightMax));
}
<file_sep>/*
Algorithm 2: Better Enumeration
Notice that in the previous algorithm,
the same sum is computed many
times. In particular, notice that Sigma(j)(k=i)a[k]
can be computed from
Sigma(j-1)(k=1)a[k] in O(1) time, rather than
starting from scratch. Write a new version
of the first algorithm that takes advantage
of this observation
*/
#include <stdio.h>
int betterEnumMaxSubarray(int a[], int n){
//counters
int i, j; //1st term, curr term
//don't need last term, go to the end every run
//variables to store arrays
/* array variables here */
//sum variables
int sum = 0;
int best = 0;
for (i = 0; i < n; i++){
sum = 0; //new starting term, new sum
for (j = i; j < n; j++){
sum += a[j];
if (sum > best){
best = sum; //new winner
/*
TODO copy array segment here maybe
*/
}
}
}
return best;
}
<file_sep>//tester.c
#include "a1.c"
#include "a2.c"
#include "a3.c"
#include <time.h>
int main(){
clock_t start, end;
double clockTime;
int numElements[] = {100, 200, 300, 400, 500,
600, 700, 800, 900,
1000, 2000, 3000, 4000, 5000,
6000, 7000, 8000, 9000};
int elementArray[9000];
int i;
int sum;
srand ( time(NULL) );
//loop to populate elementArray
for (i=0; i < 9000; i++){
elementArray[i] = (rand() % 100)-50;
}
//testing enumeration
printf("enumeration\n");
printf("elements\ttime\tsum\n");
for (i = 0; i < 9; i++){
start = clock();
sum = enumMaxSubarray(elementArray, numElements[i]);
end = clock();
clockTime = ((double) (end - start))/CLOCKS_PER_SEC;
printf("%d\t%f\t%d\n",numElements[i], clockTime, sum );
}
//testing better enumeration
printf("\n\nbetter enumeration\n");
printf("elements\ttime\tsum\n");
for (i = 0; i < 18; i++){
start = clock();
sum = betterEnumMaxSubarray(elementArray, numElements[i]);
end = clock();
clockTime = ((double) (end - start))/CLOCKS_PER_SEC;
printf("%d\t%f\t%d\n",numElements[i], clockTime, sum );
}
//testing divide and conquer
printf("\n\ndivide and conquer\n");
printf("elements\ttime\tsum\n");
for (i = 0; i < 18; i++){
start = clock();
sum = divAndConMaxSubarray(elementArray,0, numElements[i]);
end = clock();
clockTime = ((double) (end - start))/CLOCKS_PER_SEC;
printf("%d\t%f\t%d\n",numElements[i], clockTime, sum );
}
/* stuff goes here */
/* old shit
int testArray[] = {-18, -47, -40, -43, -2, 48, 31, -24, 36, -49, 4, -29, -4, -39, 12, 24, 8, 40, 45, -17, 6, -11, -5, -30, -8, 25, -44, -9, -20, -50, -12, -32, 41, 10, -42, -15, 11, -38, 37, 21, 33, -22, 16, -41, -46, -33, -26, 7, -3, -28, 29, 20, 27, 3, 15, 49, 23, -1, 14, 32, -31, -13, -48, -14, 13, 39, 46, -35, -36, 0, 17, -27, -21, 28, -7, 44, -10, 34, -19, 47, 42, -34, 5, 26, -45, 35, 9, -25, 38, -37, -23, 22, -6, -16, 18, 43, 30, 2, 19, 1};
int length = 100;
int sum = 0;
sum = divAndConMaxSubarray(testArray, 0, length);
end = clock();
clockTime = ((double) (end - start))/CLOCKS_PER_SEC;
printf("sum = %d\n",sum);
printf("clock time %d", clockTime);
*/
//
//loop to create giant array
return 0;
} | da6daa93e6a4273b72c6d120ea2095e2a1ab4104 | [
"Markdown",
"C"
] | 5 | C | samuelsnyder/max-sum-sub-array | e39cd3fdb7eafd0d1b90b69926387c568cf7a888 | 25296a2f8f48d3ce8c8f51ee24d512ec6d845ca5 | |
refs/heads/master | <file_sep>
/*
RC Base
3/12/17 by <NAME> and <NAME>
(Based on Code by <NAME>)
Allows for driving the test robot using an RC controller
*/
#include <Servo.h>
// Create Variables to hold the Receiver signals
int Ch1, Ch2, Ch3, Ch4, Ch5, Ch6;
int CalcHold; //Variable to temp hold calculations for steering stick corections
int Lwheel;
int Rwheel;
// Create Servo Objects as defined in the Servo.h files
Servo R_Servo; // Servo DC Motor Driver (Designed for RC cars)Servo R_Servo; // Servo DC Motor Driver (Designed for RC cars)
Servo L_Servo; // Servo DC Motor Driver (Designed for RC cars)Servo L_Servo; // Servo DC Motor Driver (Designed for RC cars)
int L_PhotoValue; // Variable to hold Left photo data
int R_PhotoValue; // Variable to hold Right photo data
int SharpValue; //Variable to hold prox sensor data
const int L_PhotoPin = A9; //Pin connecting the left photo
const int R_PhotoPin = A8; //Pin connecting the right photo
const int SharpPin = A7; //Pin connecting the sharp sensor
//**************************************************************
//***************** Setup ************************************
//**************************************************************
void setup() {
// Set the pins that the transmitter will be connected to all to input
pinMode(12, INPUT); //I connected this to Chan1 of the Receiver
pinMode(11, INPUT); //I connected this to Chan2 of the Receiver
pinMode(10, INPUT); //I connected this to Chan3 of the Receiver
pinMode(9, INPUT); //I connected this to Chan4 of the Receiver
pinMode(8, INPUT); //I connected this to Chan5 of the Receiver
pinMode(7, INPUT); //I connected this to Chan6 of the Receiver
pinMode(13, OUTPUT); //Onboard LED to output for diagnostics
// Attach Speed controller that acts like a servo to the board
L_Servo.attach(3); //Pin 2
R_Servo.attach(2); //Pin 3
//Flash the LED on and Off 10x Start
for (int i = 0; i < 10; i++) {
digitalWrite(13, HIGH);
delay(100);
digitalWrite(13, LOW);
delay(100);
}
//Flash the LED on and Off 10x End
Serial.begin(9600);
}
//********************** mixLimits() ***************************
//******* Make sure values never exceed ranges ***************
//****** For most all servos and like controlers *************
//**** control must fall between 1000uS and 2000uS **********
//**************************************************************
void setLimits() {
if (Rwheel < 1000) {// Can be set to a value you don't wish to exceed
Rwheel = 1000; // to adjust maximums for your own robot
}
if (Rwheel > 2000) {// Can be set to a value you don't wish to exceed
Rwheel = 2000; // to adjust maximums for your own robot
}
if (Lwheel < 1000) {// Can be set to a value you don't wish to exceed
Lwheel = 1000; // to adjust maximums for your own robot
}
if (Lwheel > 2000) {// Can be set to a value you don't wish to exceed
Lwheel = 2000; // to adjust maximums for your own robot
}
pulseMotors();
//L_Servo.writeMicroseconds(Lwheel);
//R_Servo.writeMicroseconds(Rwheel);
// printWheelCalcs(); //REMEMBER: printing values slows reaction times
}
//***************** printWheelCalcs() ************************
//******* Prints calculated wheel output values **************
//**************************************************************
void printWheelCalcs() {
Serial.print("Lwheel = ");
Serial.println(Lwheel);
Serial.print("Rwheel = ");
Serial.println(Rwheel);
}
//********************** printChannels() ***************************
//*** Simply print the collected RC values for diagnostics ***
//**************************************************************
void printChannels()
{ // print out the values you read in:
Serial.println(" RC Control Mode ");
Serial.print("Value Ch1 = ");
Serial.println(Ch1);
Serial.print("Value Ch2 = ");
Serial.println(Ch2);
}
//******************** testWheels() **************************
//* Direct call to Servos to test wheel speed and direction **
//**************************************************************
void testWheels() {
L_Servo.writeMicroseconds(1500); // 1000-2000, 1500 should be stop
R_Servo.writeMicroseconds(1500); // 1000-2000, 1500 should be stop
}
//******************* pulseMotors ***************************
//pulses either mapped or direct signals generated from mixLimits
//**************************************************************
void pulseMotors() {
//un-comment the next two line to drive the wheels directly with the MaxLimits Set
//L_Servo.writeMicroseconds(Lwheel);
//R_Servo.writeMicroseconds(Rwheel);
//un-comment the next two to map a control range.
//*** Take the standard range of 1000 to 2000 and frame it to your own minimum and maximum
//*** for each wheel.
Lwheel = map(Lwheel, 1000, 2000, 1090, 1865); // >1500 is reverse
Rwheel = map(Rwheel, 1000, 2000, 1000, 2000);// >1500 is forward
L_Servo.writeMicroseconds(Lwheel);
R_Servo.writeMicroseconds(Rwheel);
// un-comment this line do display the value being sent to the motors
// printWheelCalcs(); //REMEMBER: printing values slows reaction times
}
//******************* driveServosRC() ************************
//****** Use the value collected from Ch1 and Ch2 ************
//****** on a single stick to relatively calculate ***********
//**** speed and direction of two servo driven wheels *********
//**************************************************************
void driveServosRC()
{
if (Ch2 <= 1500) {
Rwheel = Ch1 + Ch2 - 1500;
Lwheel = Ch1 - Ch2 + 1500;
setLimits();
}
if (Ch2 > 1500) {
int Ch1_mod = map(Ch1, 1000, 2000, 2000, 1000); // Invert the Ch1 axis to keep the math similar
Rwheel = Ch1_mod + Ch2 - 1500;
Lwheel = Ch1_mod - Ch2 + 1500;
setLimits();
}
}
//***************** spin() ************************
//******* Rotate on a Dime **************
//**************************************************************
void spin(int t) {
Lwheel = 1500+t;
Rwheel = 1500+t;
pulseMotors();
}
//***************** brake() ************************
//******* Stop the robot and pause **************
//**************************************************************
void brake() {
Lwheel = 1500;
Rwheel = 1500;
pulseMotors();
delay(1000);
}
//***************** straightFwd() ************************
//******* Drive straight **************
//**************************************************************
void straightFwd(int d) {
Lwheel = 1500-d - 20;
Rwheel = 1500+d -25 ;
pulseMotors();
}
//***************** straightRev() ************************
//******* Drive straight **************
//**************************************************************
void straightRev(int d) {
Lwheel = 1500+d;
Rwheel = 1500-d;
pulseMotors();
}
//***************** driveLeft() ************************
//******* Drive left - speed determined by d rotation by t **************
//**************************************************************
void driveLeft(int d, int t) {
Lwheel = 1500-d -20;
Rwheel = 1500+d+t -27;
pulseMotors();
}
//***************** driveRight() ************************
//******* Drive right - speed determined by d rotation by t **************
//**************************************************************
void driveRight(int d, int t) {
Lwheel = 1500-d-t -20;
Rwheel = 1500+d -25;
pulseMotors();
}
//***************** navToLight() ************************
//******* Find light and drive to it until you are 1ft away **************
//**************************************************************
void navToLight() {
int const DELTA = 300; //If -DELTA<Delta<DELTA you are looking straight at the light or at ambient
int AMB = 800; //Sensor is looking at ambient if reading this or higher
L_PhotoValue = analogRead(L_PhotoPin); //Read the value of the photo sensor
R_PhotoValue = analogRead(R_PhotoPin); //Read the value of the photo sensor
SharpValue = analogRead(SharpPin);
int Delta = L_PhotoValue - R_PhotoValue;
//You are one foot away
if(SharpValue > 200){
brake();
return;
}
//Light is out of range
if(Delta<DELTA && Delta>(-DELTA) && (L_PhotoValue>AMB || R_PhotoValue>AMB)){
spin(55);
}
//Too close to box or obstacle - back up
if(SharpValue >= 400){
straightRev(100);
}
//Light is straight ahead
if(Delta<DELTA && Delta>(-DELTA) && (L_PhotoValue<AMB || R_PhotoValue<AMB)){
straightFwd(90);
}
//Light is to the left
if(Delta<(-DELTA)){
driveLeft(90, 5);
}
//Light is to the right
if(Delta>DELTA){
driveRight(90, 5);
}
}
//************************ loop() ****************************
//********************** Main Loop ***************************
//**************************************************************
void loop()
{
navToLight();
}
<file_sep># Autonomous_Light_Tracking
This repository contains code for navigating to a light source autonomously. The code also includes RC capabilities.
| 15bcddcd09d26fdf453d0998df65eb01beea879c | [
"Markdown",
"C++"
] | 2 | C++ | TeamWoahBot/Autonomous_Light_Tracking | bf4d51cad8163f69c102267c7986d97f05c44ab3 | 7ba03a94cd36da3a7f0e51bf2b94bc677a314fce | |
refs/heads/master | <repo_name>XenoEmblems/lynda<file_sep>/Ex_Files_FoP_Fun/Exercise Files/ch04/Complex Conditions/script.js
var balance = 5000;
if ( balance > 0 ) {
alert("The balance is positive");
if ( balance > 10000 ) {
alert("The balance is large!");
}
} else if (balance == 0) {
alert("There is no balance");
} else {
alert("The balance is negative");
} | a9dd4a174710796af1049f97695533d77b9e5716 | [
"JavaScript"
] | 1 | JavaScript | XenoEmblems/lynda | 44b3fc8b2b810fbc2af6fed690443060d9ed0064 | dcf32ea965c9f109fb07f889d041086c0f0a0e98 | |
refs/heads/main | <repo_name>ccccc-ccccc/test<file_sep>/lm_bbdinf.cpp
#include "lm_bbdinf.hpp"
/*
function: crc checking and deconverging without 8to32
idata[i]
finf[o]
odata[o]
*/
void lm_bbdchk(stream<U8> &idata, stream<lm_frm_info> &finf, stream<U8> &odata)
{
#pragma HLS pipeline II=1 enable_flush
static enum enState {RS_NIL = 0, RS_1TYPE, RS_AFT1TYPE, RS_TYPE, RS_AFTTYPE, RS_LEN1, RS_LEN2, RS_RXING, RS_TRXING, RS_PADING, RS_CRC1, RS_CRC2} rfs;
static CRC_VAL cval, ccval;
static ap_uint<12> rbc, fstp, fedp;
static ap_uint<3> frms;
static ap_uint<7> ftyp;
static ap_uint<1> nh;
static ap_uint<8> send;
static ap_uint<3> cnt;
static ap_uint<12> flen;
static lm_frm_info info;
#pragma HLS ARRAY_PARTITION variable=info.flen complete dim=1
#pragma HLS ARRAY_PARTITION variable=info.fpos complete dim=1
#pragma HLS ARRAY_PARTITION variable=info.ftyp complete dim=1
static U32 tick;
static U32 fttag;
switch(rfs) {
case RS_NIL:
fedp = 0;
cval = 0;
ccval = 0;
frms = 0;
fstp = 0;
cnt = 0;
if (false == idata.empty()) {
U8 val8;
idata.read(val8);
lm_calcrc_8toV(val8, ccval);
odata.write(val8);
info.send = val8;
rfs = RS_1TYPE;
rbc = 1;
/* record time tag */
fttag = tick;
lm_clrxinfo(info);
}
break;
case RS_1TYPE:
if (false == idata.empty()) {
info.ttag = fttag;
U8 val8, tmp;
idata.read(val8);
lm_calcrc_8toV(val8, ccval);
tmp = val8(6, 0);
odata.write(tmp);
ftyp = val8(6, 0);
nh = val8(7,7);
lm_ftp2len(ftyp, flen);
rfs = RS_AFT1TYPE;
}
break;
case RS_AFT1TYPE: {
if (flen > 0) {
info.ftyp[frms] = ftyp;
info.fpos[frms] = 0;
info.flen[frms] = flen + 1;
fstp = 0;
fedp = flen;
if (1 == nh) {
rfs = RS_RXING;
}
else {
rfs = RS_TRXING;
}
}
else {
info.ftyp[frms] = ftyp;
info.fpos[frms] = 0;
fstp = 0;
flen = 2;
cnt = 1;
rfs = RS_LEN1;
}
rbc += 1;
break;
}
case RS_TYPE:
if (false == idata.empty()) {
U8 val8, tmp;
idata.read(val8);
lm_calcrc_8toV(val8, ccval);
tmp = val8(6, 0);
odata.write(tmp);
ftyp = val8(6, 0);
nh = val8(7,7);
lm_ftp2len(ftyp, flen);
rfs = RS_AFTTYPE;
}
break;
case RS_AFTTYPE: {
if (flen > 0) {
info.ftyp[frms] = ftyp;
info.fpos[frms] = rbc;
info.flen[frms] = flen;
fstp = rbc;
fedp = rbc + flen - 1;
if (1 == nh) {
rfs = RS_RXING;
}
else {
rfs = RS_TRXING;
}
}
else {
info.ftyp[frms] = ftyp;
info.fpos[frms] = rbc;
fstp = rbc;
flen = 1;
cnt = 1;
rfs = RS_LEN1;
}
rbc += 1;
break;
}
case RS_LEN1:
if (false == idata.empty()) {
U8 val8;
idata.read(val8);
lm_calcrc_8toV(val8, ccval);
odata.write(val8);
if (cnt == 7) {
/* 1-byte length */
if (0 == val8(7, 7)) {
info.flen[frms] = flen + val8(6, 0);
fedp = fstp + flen + val8(6, 0) - 1;
if (1 == nh) {
rfs = RS_RXING;
}
else {
rfs = RS_TRXING;
}
}
/* 2-byte length */
else {
ap_uint<12> tmp;
tmp = val8(3,0);
flen += tmp<<8;
rfs = RS_LEN2;
}
}
else {
flen += 1;
cnt += 1;
}
rbc += 1;
}
break;
case RS_LEN2:
if (false == idata.empty()) {
U8 val8;
idata.read(val8);
lm_calcrc_8toV(val8, ccval);
odata.write(val8);
flen += val8;
info.flen[frms] = flen;
fedp = fstp + flen - 1;
if (1 == nh) {
rfs = RS_RXING;
}
else {
rfs = RS_TRXING;
}
rbc += 1;
}
break;
case RS_RXING:
if (false == idata.empty()) {
U8 val8;
idata.read(val8);
lm_calcrc_8toV(val8, ccval);
odata.write(val8);
if (rbc == fedp) {
frms += 1;
info.cfrm += 1;
rfs = RS_TYPE;
}
rbc += 1;
}
break;
case RS_TRXING:
if (false == idata.empty()) {
U8 val8;
idata.read(val8);
lm_calcrc_8toV(val8, ccval);
odata.write(val8);
if (fedp == rbc) {
frms += 1;
info.cfrm += 1;
info.tlen = rbc + 1;
if (rbc + 1 == MR_BB_MTU - MR_CRC_LEN) {
rfs = RS_CRC1;
}
else {
rfs = RS_PADING;
}
}
rbc += 1;
}
break;
case RS_PADING:
if (false == idata.empty()) {
U8 val8;
idata.read(val8);
rbc += 1;
if (rbc == MR_BB_MTU - MR_CRC_LEN) {
rfs = RS_CRC1;
}
}
break;
case RS_CRC1:
if (false == idata.empty()) {
U8 val8;
idata.read(val8);
rbc += 1;
cval(7, 0) = val8;
rfs = RS_CRC2;
}
break;
case RS_CRC2:
if (false == idata.empty()) {
U8 val8;
idata.read(val8);
rbc += 1;
cval(15, 8) = val8;
if (cval == ccval) {
info.gfrm = 1;
finf.write(info);
#ifndef __SYNTHESIS__
short fcnt = info.cfrm;
short alen = info.tlen;
UW16 crc = cval;
printf("[crc]: a good cvg frame, count=%d, length=%d, crc=%x\n", fcnt, alen, crc);
#endif
}
else {
info.gfrm = 0;
finf.write(info);
#ifndef __SYNTHESIS__
unsigned short crc1, crc2;
crc1 = cval;
crc2 = ccval;
printf("[crc]: drop a bad frame due to incorrect crc, ccval=%x, cval=%x\n", crc2, crc1);
#endif
}
rfs = RS_NIL;
}
break;
}
tick = tick + 1;
// UW64 tmp = tick;
// printf("tick_bb=%d\n",tmp);
}
/*
function: bb data input, 8to32 and dispatch to different module
*/
void lm_bbdproc(stream<lm_frm_info> &finf, stream<U8> &rfrm, stream<lm_idu_dwd> &dfrm, stream<lm_idu_dwd> &sfrm, stream<lm_idu_dwd> &cfrm)
{
#pragma HLS pipeline II=1 enable_flush
static ap_uint<12> rbc, flen;
static U8 type;
static ap_uint<2> bpos;
static ap_uint<3> fidx;
static lm_idu_dwd cword;
static lm_frm_info inf;
#pragma HLS ARRAY_PARTITION variable=inf.flen complete dim=1
#pragma HLS ARRAY_PARTITION variable=inf.fpos complete dim=1
#pragma HLS ARRAY_PARTITION variable=inf.ftyp complete dim=1
static enum enState {BPS_NIL = 0, BPS_DISP, BPS_DFWDING, BPS_SFWDING, BPS_CFWDING, BPS_NEWFRM, BPS_DROPPING} bbps;
switch(bbps) {
case BPS_NIL: {
if (false == finf.empty()) {
if (false == rfrm.empty()) {
fidx = 0;
rbc = 0;
finf.read(inf);
/* forward */
if (1 == inf.gfrm) {
#ifndef __SYNTHESIS__
unsigned short num[4];
num[0] = inf.ftyp[fidx];
num[1] = inf.fpos[fidx];
num[2] = inf.flen[fidx];
num[3] = rbc;
EPT2("[fd]: new frame, type=%d, pos=%d, len=%d, rbc=%d\n", num[0], num[1], num[2], num[3]);
#endif
type = inf.ftyp[fidx];
cword.data(15, 0) = 4 + inf.flen[fidx];
cword.data(19, 16) = 1; // rx frames
cword.data(31, 20) = inf.ttag(11, 0); // time tag
cword.last = 0;
bpos = 0;
bbps = BPS_DISP;
}
/* drop */
else {
U8 val8;
rfrm.read(val8);
rbc += 1;
bbps = BPS_DROPPING;
}
}
}
break;
}
case BPS_DISP:
if (type < PKT2_COD_BASE) {
if (type == PKT_LNK_SERFB || type == PKT_LNK_SERFS) {
cfrm.write(cword);
sfrm.write(cword);
bbps = BPS_SFWDING;
}
else {
cfrm.write(cword);
bbps = BPS_CFWDING;
}
}
else if (type < PKT2_LBL_BASE) {
EPT_HERE;
dfrm.write(cword);
bbps = BPS_DFWDING;
}
else {
U8 val8;
rfrm.read(val8);
rbc += 1;
bbps = BPS_DROPPING;
}
break;
case BPS_DROPPING: {
if (false == rfrm.empty()) {
U8 val8;
rfrm.read(val8);
rbc += 1;
if (rbc == inf.tlen) {
bbps = BPS_NIL;
}
}
break;
}
case BPS_DFWDING: {
if (false == rfrm.empty()) {
U8 val8;
rfrm.read(val8);
rbc += 1;
bpos += 1;
flen += 1;
lm_byte2dword(bpos, val8, cword.data);
if (flen == inf.flen[fidx]) {
cword.last = 1;
dfrm.write(cword);
if (rbc != inf.tlen) {
fidx += 1;
bbps = BPS_NEWFRM;
}
else {
bbps = BPS_NIL;
}
}
else if (bpos == 0) {
dfrm.write(cword);
}
}
break;
}
case BPS_SFWDING: {
if (false == rfrm.empty()) {
U8 val8;
rfrm.read(val8);
rbc += 1;
bpos += 1;
flen += 1;
lm_byte2dword(bpos, val8, cword.data);
if (flen == inf.flen[fidx]) {
#ifndef __SYNTHESIS__
EPT2("[fd]: end of dispatching a new sfm.\n");
#endif
cword.last = 1;
cfrm.write(cword);
sfrm.write(cword);
if (rbc != inf.tlen) {
fidx += 1;
bbps = BPS_NEWFRM;
}
else {
bbps = BPS_NIL;
}
}
else if (bpos == 0) {
cfrm.write(cword);
sfrm.write(cword);
}
}
break;
}
case BPS_CFWDING: {
if (false == rfrm.empty()) {
U8 val8;
rfrm.read(val8);
rbc += 1;
bpos += 1;
flen += 1;
lm_byte2dword(bpos, val8, cword.data);
if (flen == inf.flen[fidx]) {
cword.last = 1;
cfrm.write(cword);
if (rbc != inf.tlen) {
fidx += 1;
bbps = BPS_NEWFRM;
}
else {
bbps = BPS_NIL;
}
}
else if (bpos == 0) {
cfrm.write(cword);
}
}
break;
}
case BPS_NEWFRM: {
lm_idu_dwd tword;
#ifndef __SYNTHESIS__
unsigned short num[4];
num[0] = inf.ftyp[fidx];
num[1] = inf.fpos[fidx];
num[2] = inf.flen[fidx];
num[3] = rbc;
EPT2("[fd]: new frame, type=%d, pos=%d, len=%d, rbc=%d\n", num[0], num[1], num[2], num[3]);
#endif
/* add send node */
type = inf.ftyp[fidx];
flen = 0;
bpos = 1;
cword.data(7, 0) = inf.send;
cword.last = 0;
/* add frame length, send node */
tword.data(15, 0) = 4 + inf.flen[fidx] + 1;
tword.data(19, 16) = 1; // rx frame
tword.data(31, 20) = inf.ttag(11, 0); // time tag
tword.last = 0;
if (type < PKT2_COD_BASE) {
if (type == PKT_LNK_SERFB || type == PKT_LNK_SERFS) {
cfrm.write(tword);
sfrm.write(tword);
bbps = BPS_SFWDING;
}
else {
cfrm.write(tword);
bbps = BPS_CFWDING;
}
}
else if (type < PKT2_LBL_BASE) {
dfrm.write(tword);
bbps = BPS_DFWDING;
}
else {
U8 val8;
rfrm.read(val8);
rbc += 1;
bbps = BPS_DROPPING;
}
}
}
}
/*
function: frame filter
ifrm[i]
icmd[i]
vld[o]
ofrm[o]
*/
void lm_frmfilter(stream<lm_idu_dwd> &icmd, stream<lm_idu_dwd> &ifrm, stream<T_SIG_EN> &vld, stream<lm_idu_dwd> &ofrm)
{
#pragma HLS pipeline II=1 enable_flush
static enum enState {RC_NIL = 0, RC_IDLE, RC_SASET, RC_LOOP} rcs;
static ap_uint<2> sflg;
static ap_uint<12> cfl;
static U8 sa;
static U8 sa_bk;
static enum enFFState {FF_NIL = 0, FF_DROP, FF_TYPE, FF_ACMP, FF_ING} ffs;
static ap_uint<12> dlen;
switch(rcs) {
case RC_NIL:
if (false == icmd.empty()) {
lm_idu_dwd value;
icmd.read(value);
cfl = value.data(11, 0);
rcs = RC_SASET;
}
break;
case RC_IDLE:
if (false == icmd.empty()) {
lm_idu_dwd value;
icmd.read(value);
cfl = value.data(11, 0);
rcs = RC_SASET;
}
break;
case RC_SASET:
if (false == icmd.empty()) {
lm_idu_dwd value;
icmd.read(value);
if (sflg == 0) {
sa = value.data(7, 0);
sflg = 1;
}
else {
sa_bk = value.data(7, 0);
sflg = 2;
}
if (value.last == 1) {
rcs = RC_IDLE;
}
else {
rcs = RC_LOOP;
}
}
break;
case RC_LOOP:
if (false == icmd.empty()) {
lm_idu_dwd value;
icmd.read(value);
if (value.last == 1) {
rcs = RC_IDLE;
}
}
break;
}
switch(ffs) {
case FF_NIL:
if (false == ifrm.empty()) {
lm_idu_dwd value;
ifrm.read(value);
dlen = value.data(11, 0);
ofrm.write(value);
ffs = FF_TYPE;
}
else if (sflg == 2) {
sa = sa_bk;
sflg = 1;
}
break;
case FF_TYPE:
if (false == ifrm.empty()) {
lm_idu_dwd value;
ifrm.read(value);
ofrm.write(value);
ffs = FF_ACMP;
}
else if (sflg == 2) {
sa = sa_bk;
sflg = 1;
}
break;
case FF_ACMP:
if (false == ifrm.empty()) {
if (sflg == 0) {
lm_idu_dwd value;
ifrm.read(value);
value.last = 1;
ofrm.write(value);
vld.write(0);
ffs = FF_DROP;
}
else {
lm_idu_dwd value;
ifrm.read(value);
U8 recv;
recv = value.data(7, 0);
if (true == lm_adfilter(sa, recv)) {
ofrm.write(value);
vld.write(1);
ffs = FF_ING;
}
else {
value.last = 1;
ofrm.write(value);
vld.write(0);
ffs = FF_DROP;
}
}
}
break;
case FF_ING:
if (false == ifrm.empty()) {
lm_idu_dwd value;
ifrm.read(value);
ofrm.write(value);
if (value.last == 1) {
ffs = FF_NIL;
}
}
break;
case FF_DROP:
if (false == ifrm.empty()) {
lm_idu_dwd value;
ifrm.read(value);
if (value.last == 1) {
ffs = FF_NIL;
}
}
else if (sflg == 2) {
sa = sa_bk;
sflg = 1;
}
break;
}
}
bool lm_adfilter(U8 sa, U8 recv)
{
bool rval = false;
if (recv == sa || recv == MR_BCAST_ADD) {
rval = true;
}
return rval;
}
void lm_calcrc_8toV(U8 bval, CRC_VAL &crc)
{
static const unsigned short Crc16Table[256] =
{
0x0000, 0xC0C1, 0xC181, 0x0140, 0xC301, 0x03C0, 0x0280, 0xC241,
0xC601, 0x06C0, 0x0780, 0xC741, 0x0500, 0xC5C1, 0xC481, 0x0440,
0xCC01, 0x0CC0, 0x0D80, 0xCD41, 0x0F00, 0xCFC1, 0xCE81, 0x0E40,
0x0A00, 0xCAC1, 0xCB81, 0x0B40, 0xC901, 0x09C0, 0x0880, 0xC841,
0xD801, 0x18C0, 0x1980, 0xD941, 0x1B00, 0xDBC1, 0xDA81, 0x1A40,
0x1E00, 0xDEC1, 0xDF81, 0x1F40, 0xDD01, 0x1DC0, 0x1C80, 0xDC41,
0x1400, 0xD4C1, 0xD581, 0x1540, 0xD701, 0x17C0, 0x1680, 0xD641,
0xD201, 0x12C0, 0x1380, 0xD341, 0x1100, 0xD1C1, 0xD081, 0x1040,
0xF001, 0x30C0, 0x3180, 0xF141, 0x3300, 0xF3C1, 0xF281, 0x3240,
0x3600, 0xF6C1, 0xF781, 0x3740, 0xF501, 0x35C0, 0x3480, 0xF441,
0x3C00, 0xFCC1, 0xFD81, 0x3D40, 0xFF01, 0x3FC0, 0x3E80, 0xFE41,
0xFA01, 0x3AC0, 0x3B80, 0xFB41, 0x3900, 0xF9C1, 0xF881, 0x3840,
0x2800, 0xE8C1, 0xE981, 0x2940, 0xEB01, 0x2BC0, 0x2A80, 0xEA41,
0xEE01, 0x2EC0, 0x2F80, 0xEF41, 0x2D00, 0xEDC1, 0xEC81, 0x2C40,
0xE401, 0x24C0, 0x2580, 0xE541, 0x2700, 0xE7C1, 0xE681, 0x2640,
0x2200, 0xE2C1, 0xE381, 0x2340, 0xE101, 0x21C0, 0x2080, 0xE041,
0xA001, 0x60C0, 0x6180, 0xA141, 0x6300, 0xA3C1, 0xA281, 0x6240,
0x6600, 0xA6C1, 0xA781, 0x6740, 0xA501, 0x65C0, 0x6480, 0xA441,
0x6C00, 0xACC1, 0xAD81, 0x6D40, 0xAF01, 0x6FC0, 0x6E80, 0xAE41,
0xAA01, 0x6AC0, 0x6B80, 0xAB41, 0x6900, 0xA9C1, 0xA881, 0x6840,
0x7800, 0xB8C1, 0xB981, 0x7940, 0xBB01, 0x7BC0, 0x7A80, 0xBA41,
0xBE01, 0x7EC0, 0x7F80, 0xBF41, 0x7D00, 0xBDC1, 0xBC81, 0x7C40,
0xB401, 0x74C0, 0x7580, 0xB541, 0x7700, 0xB7C1, 0xB681, 0x7640,
0x7200, 0xB2C1, 0xB381, 0x7340, 0xB101, 0x71C0, 0x7080, 0xB041,
0x5000, 0x90C1, 0x9181, 0x5140, 0x9301, 0x53C0, 0x5280, 0x9241,
0x9601, 0x56C0, 0x5780, 0x9741, 0x5500, 0x95C1, 0x9481, 0x5440,
0x9C01, 0x5CC0, 0x5D80, 0x9D41, 0x5F00, 0x9FC1, 0x9E81, 0x5E40,
0x5A00, 0x9AC1, 0x9B81, 0x5B40, 0x9901, 0x59C0, 0x5880, 0x9841,
0x8801, 0x48C0, 0x4980, 0x8941, 0x4B00, 0x8BC1, 0x8A81, 0x4A40,
0x4E00, 0x8EC1, 0x8F81, 0x4F40, 0x8D01, 0x4DC0, 0x4C80, 0x8C41,
0x4400, 0x84C1, 0x8581, 0x4540, 0x8701, 0x47C0, 0x4680, 0x8641,
0x8201, 0x42C0, 0x4380, 0x8341, 0x4100, 0x81C1, 0x8081, 0x4040
};
#pragma HLS ARRAY_PARTITION variable=Crc16Table complete dim=1
//开始计算CRC16校验值
unsigned short TableNo=0;
TableNo=((crc & 0xff)^(bval & 0xff));
crc=((crc>>8)&0xff)^Crc16Table[TableNo];
}
void lm_calcrc_32toV(ap_uint<3> cnt, U32 bval, CRC_VAL &crc)
{
crc = 0x5a5a;
}
#if 1
void lm_ftp2len(U8 ftype, ap_uint<12> &len)
{
#pragma HLS INLINE
const static ap_uint<8> lkpl[] = {
31, 17, 1, 1, 2, 6, 0, 0, 5
};
#pragma HLS ARRAY_PARTITION variable=lkpl complete dim=1
if (ftype < PKT2_COD_BASE) {
len = lkpl[ftype];
}
else {
len = 0;
}
}
#else
void lm_ftp2len(U8 ftype, ap_uint<12> &len)
{
#pragma HLS INLINE
/* const static ap_uint<8> lkpl[] = {
25, 17, 1, 1, 2, 6, 0, 0, 5
};
*/
switch(ftype) {
case 0:
len = 25;
break;
case 1:
len = 17;
break;
case 2:
len = 1;
break;
case 3:
len = 1;
break;
case 4:
len = 2;
break;
case 5:
len = 6;
break;
case 6:
len = 0;
break;
case 7:
len = 0;
break;
case 8:
len = 5;
break;
default:
len = 0;
break;
}
}
#endif
void lm_byte2dword(ap_uint<2> pos, U8 val8, U32 &val32)
{
#pragma HLS INLINE
if (pos == 1) {
val32(7, 0) = val8;
UW8 tmp = val32(7, 0);
// printf("cword=%x\n",tmp);
}
else if (pos == 2) {
val32(15, 8) = val8;
UW8 tmp = val32(15, 8);
// printf("cword=%x\n",tmp);
}
else if (pos == 3) {
val32(23, 16) = val8;
UW8 tmp = val32(23, 16);
// printf("cword=%x\n",tmp);
}
else {
val32(31, 24) = val8;
UW8 tmp = val32(31, 24);
// printf("cword=%x\n",tmp);
}
}
void lm_clrxinfo(lm_frm_info &rinf)
{
rinf.cfrm = 0;
rinf.gfrm = 0;
rinf.tlen = 0;
for (int i = 0; i < LM_CFRM_CNT; ++i) {
rinf.flen[i] = 0;
rinf.fpos[i] = 0;
rinf.ftyp[i] = 0;
}
}
void lm_btxdata(stream<lm_bbt_dwd> &idata, stream<U8> &odata)
{
#pragma HLS pipeline II=1 enable_flush
static enum enState {BTS_NIL = 0, BTS_BYTE0, BTS_BYTE1, BTS_BYTE2, BTS_BYTE3, BTS_FEND, BTS_PADDING, BTS_CRC1, BTS_CRC2} bts;
static ap_uint<10> plen;
static lm_bbt_dwd tword;
static U8 bval;
static U16 cval;
switch(bts) {
case BTS_NIL:
if (false == idata.empty()) {
idata.read(tword);
bval = tword.data(7, 0);
odata.write(bval);
lm_calcrc_8toV(bval, cval);
cval = 0;
plen = 1;
bts = BTS_BYTE1;
}
break;
case BTS_BYTE0:{
idata.read(tword);
bval = tword.data(7, 0);
odata.write(bval);
lm_calcrc_8toV(bval, cval);
plen = plen + 1;
if (3 == tword.ivbs) {
bts = BTS_FEND;
}
else {
bts = BTS_BYTE1;
}
break;
}
case BTS_BYTE1: {
bval = tword.data(15, 8);
odata.write(bval);
lm_calcrc_8toV(bval, cval);
plen = plen + 1;
if (2 == tword.ivbs) {
bts = BTS_FEND;
}
else {
bts = BTS_BYTE2;
}
break;
}
case BTS_BYTE2: {
bval = tword.data(23, 16);
odata.write(bval);
lm_calcrc_8toV(bval, cval);
plen = plen + 1;
if (1 == tword.ivbs) {
bts = BTS_FEND;
}
else {
bts = BTS_BYTE3;
}
break;
}
case BTS_BYTE3: {
bval = tword.data(31, 24);
odata.write(bval);
lm_calcrc_8toV(bval, cval);
plen = plen + 1;
if (1 == tword.last) {
bts = BTS_FEND;
}
else {
bts = BTS_BYTE0;
}
break;
}
case BTS_FEND:{
if (plen == MR_LMIDU_PL) {
bval = cval(7, 0);
odata.write(bval);
bts = BTS_CRC2;
}
else if (plen + 1 == MR_LMIDU_PL) {
odata.write(0);
lm_calcrc_8toV(0, cval);
bts = BTS_CRC1;
}
else if (false == idata.empty()) {
idata.read(tword);
bval = tword.data(7, 0);
odata.write(bval);
lm_calcrc_8toV(bval, cval);
plen = plen + 1;
bts = BTS_BYTE1;
}
else {
odata.write(0);
lm_calcrc_8toV(0, cval);
plen = plen + 1;
bts = BTS_PADDING;
}
break;
}
case BTS_PADDING: {
odata.write(0);
lm_calcrc_8toV(0, cval);
if (plen + 1 == MR_LMIDU_PL) {
bts = BTS_CRC1;
}
else {
plen = plen + 1;
}
break;
}
case BTS_CRC1: {
bval = cval(7, 0);
odata.write(bval);
bts = BTS_CRC2;
break;
}
case BTS_CRC2: {
bval = cval(15, 8);
odata.write(bval);
#ifndef __SYNTHESIS__
printf("*****BB OUT SUCCESS!!!*****\n");
#endif
bts = BTS_NIL;
break;
}
}
}
| 6b1f395c0e5b41635a8d493e2cba09d5a228ff82 | [
"C++"
] | 1 | C++ | ccccc-ccccc/test | 816ffa5b38a0bfc22b8a6877a21bfe739ba3a363 | 92f93f6c479a68a34bbbc1191c1e02d13a0e89e9 | |
refs/heads/master | <file_sep>package sybrix.easygsp2.exceptions;
/**
* Created by dsmith on 8/16/15.
*/
public class InvokeControllerActionException extends HttpException {
public InvokeControllerActionException() {
super(500);
}
public InvokeControllerActionException(String message) {
super(message,500);
}
public InvokeControllerActionException(String message, Throwable cause) {
super(message, cause,500);
}
public InvokeControllerActionException(Throwable cause) {
super(cause,500);
}
protected InvokeControllerActionException(String message, Throwable cause, boolean enableSuppression, boolean writableStackTrace) {
super(message, cause, enableSuppression, writableStackTrace,500);
}
}
<file_sep>package sybrix.easygsp2.categories;
public class MethodSecurity {
boolean secured = false;
String [] roles = {};
public MethodSecurity(boolean secured, String[] roles) {
this.secured = secured;
this.roles = roles;
}
public boolean isSecured() {
return secured;
}
public void setSecured(boolean secured) {
this.secured = secured;
}
public String[] getRoles() {
return roles;
}
public void setRoles(String[] roles) {
this.roles = roles;
}
}
<file_sep>package sybrix.easygsp2.data;
import com.fasterxml.jackson.core.JsonProcessingException;
import sybrix.easygsp2.exceptions.SerializerException;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.lang.reflect.Type;
public interface Serializer {
public void write(Object o, OutputStream outputStream) throws SerializerException;
String toString(Object o);
Object parse(String xmlString, Class cls);
Object parse(String xmlString);
Object parse(InputStream inputStream, int length);
Object parse(InputStream inputStream, int length, Class clazz, Type modelType);
}
<file_sep>package sybrix.easygsp2.anno;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
/**
* Created by dsmith on 4/19/15.
*/
@Retention(RetentionPolicy.RUNTIME)
@Target({ElementType.METHOD, ElementType.TYPE})
public @interface Api {
String[] url();
String[] method() default {"*"};
String[] roles() default {"*"};
String[] accepts() default {};
String[] contentType() default {};
}<file_sep>package sybrix.easygsp2.util;
import javax.servlet.http.Cookie;
import javax.servlet.http.HttpServletRequest;
import java.util.Map;
import java.util.HashMap;
import java.util.Iterator;
import java.net.URLDecoder;
/**
* Created by IntelliJ IDEA.
* User: dsmith
* Date: Apr 12, 2007
* Time: 6:12:40 PM
*/
public class CookieManager {
//private static final Logger log = Logger.getLogger(CookieManager.class);
public static final int DAY = 60 * 60 * 24;
/**
* Returns an instance to the specified cookie.
*
* @param cookieName String
* @return Cookie - returns null if the cookie doesn't exists.
*/
public static Cookie getCookie(String cookieName, HttpServletRequest request) {
Cookie c[] = request.getCookies();
if (c != null) {
for (int x = 0; x < c.length; x++) {
if (c[x].getName().equals(cookieName)) {
return c[x];
}
}
}
return null;
}
public static Cookie createCookie(String cookieName, String value, int expiresInDays) {
Cookie cookie = new Cookie(cookieName, value);
cookie.setMaxAge(60 * 60 * 24 * expiresInDays);
cookie.setPath("/");
return cookie;
}
public static Map<String, String> getCookieMap(Cookie cookie, HttpServletRequest request) {
if (request.getAttribute(cookie.getName() + "_cookie") == null) {
String _value =cookie.getValue();
String pair[] = _value.split(";");
Map map = new HashMap();
for (int i = 0; i < pair.length; i++) {
int index = pair[i].indexOf('=');
if (index > -1) {
String name = pair[i].substring(0, index);
String value = pair[i].substring(index + 1, pair[i].length());
map.put(name, value);
}
}
request.setAttribute(cookie.getName() + "_cookie", map);
}
return (Map) request.getAttribute(cookie.getName() + "_cookie");
}
// public static String getCookieMapValue(Map map) {
// Iterator i = map.keySet().iterator();
// StringBuffer val = new StringBuffer(100);
// while (i.hasNext()) {
// String key = i.next().toString();
// String value = map.get(key).toString();
// if (key.trim().length() > 0)
// val.append(key).append("=").append(value).append(";");
// }
//
// Encrypt enc = new Encrypt();
// try {
// byte encrypted[] = enc.encrypt(val.toString().getBytes());
// return java.net.URLEncoder.encode(Utilities.base64Encode(encrypted),"UTF-8");
// } catch (Exception e) {
// log.error(e.getMessage(), e);
// }
//
// return "";
// }
//
// public static String decryptValue(String val) {
// Encrypt enc = new Encrypt();
// byte[] decoded = null;
// try {
// String urlDecoded = URLDecoder.decode(val,"UTF-8");
// decoded = enc.base64Decode(urlDecoded);
// byte[] decrypted = enc.decrypt(decoded);
// return new String(decrypted);
// }catch(Exception e){
// log.error(e.getMessage(),e);
// }
//
// return "";
// }
// private static byte[] padBytes(byte value[]) {
// int len = 8;
// int originalLength = value.length;
// if (value.length > len) {
// len = ((value.length / 8) + 1) * 8;
// }
//
// value = Utilities.increaseArrayLength(value, len);
//
// return value;
// }
//
// private static String pad(String value) {
// int len = 8;
// if (value.length() > len) {
// len = ((value.length() / 8) + 1) * 8;
// }
//
// StringBuffer v = new StringBuffer(len);
// v.append(value);
//
// for (int i = 0; i < len - value.length(); i++) {
// v.append(" ");
// }
//
// return v.toString();
// }
}
<file_sep>package sybrix.easygsp2;
import groovy.lang.*;
import io.jsonwebtoken.ExpiredJwtException;
import javassist.ClassPool;
import javassist.CtClass;
import javassist.CtMethod;
import javassist.LoaderClassPath;
import javassist.bytecode.CodeAttribute;
import javassist.bytecode.LocalVariableAttribute;
import org.apache.commons.fileupload.FileItem;
import org.apache.commons.io.FileUtils;
import org.codehaus.groovy.control.CompilerConfiguration;
import org.codehaus.groovy.runtime.GroovyCategorySupport;
import org.codehaus.groovy.runtime.InvokerHelper;
import org.reflections.Reflections;
import org.reflections.scanners.MethodAnnotationsScanner;
import org.reflections.scanners.SubTypesScanner;
import org.reflections.scanners.TypeElementsScanner;
import sun.reflect.generics.reflectiveObjects.ParameterizedTypeImpl;
import sybrix.easygsp2.anno.Api;
import sybrix.easygsp2.anno.Content;
import sybrix.easygsp2.anno.Secured;
import sybrix.easygsp2.categories.RoutingCategory;
import sybrix.easygsp2.controllers.JwtController;
import sybrix.easygsp2.data.Serializer;
import sybrix.easygsp2.data.XmlSerializer;
import sybrix.easygsp2.email.EmailService;
import sybrix.easygsp2.exceptions.*;
import sybrix.easygsp2.fileupload.FileUpload;
import sybrix.easygsp2.framework.*;
import sybrix.easygsp2.http.MediaType;
import sybrix.easygsp2.http.ControllerResponse;
import sybrix.easygsp2.http.MimeTypes;
import sybrix.easygsp2.models.CreateClientRequest;
import sybrix.easygsp2.routing.MethodAndRole;
import sybrix.easygsp2.routing.Route;
import sybrix.easygsp2.routing.Routes;
import sybrix.easygsp2.routing.UrlParameter;
import sybrix.easygsp2.security.*;
import sybrix.easygsp2.templates.RequestError;
import sybrix.easygsp2.templates.TemplateInfo;
import sybrix.easygsp2.templates.TemplateWriter;
import sybrix.easygsp2.util.PropertiesFile;
import javax.activation.MimeType;
import javax.activation.MimeTypeParseException;
import javax.servlet.*;
import javax.servlet.http.Cookie;
import javax.servlet.http.HttpServletResponse;
import java.beans.BeanInfo;
import java.beans.Introspector;
import java.beans.PropertyDescriptor;
import java.io.*;
import java.lang.annotation.Annotation;
import java.lang.reflect.*;
import java.net.MalformedURLException;
import java.net.URISyntaxException;
import java.net.URL;
import java.security.Principal;
import java.util.*;
import java.util.regex.Pattern;
import javax.servlet.http.HttpServletRequest;
import javax.validation.ConstraintViolation;
import javax.validation.Validation;
import javax.validation.Validator;
import javax.validation.ValidatorFactory;
import org.slf4j.LoggerFactory;
import sybrix.easygsp2.util.StringUtil;
import static sybrix.easygsp2.util.StringUtil.combine;
/**
* Created by dsmith on 7/19/16.
*/
public class EasyGsp2 {
private static final org.slf4j.Logger logger = LoggerFactory.getLogger(EasyGsp2.class);
private static final String JSON_SERIALIZER_CLASS = "sybrix.easygsp2.data.JsonSerializerImpl";
private static final String XML_SERIALIZER_CLASS = "sybrix.easygsp2.data.XmlSerializerImpl";
public static final String ROUTE_REQUEST_ATTR = "__route__";
public static final MimeType APPLICATION_JSON = parseMimeType("application/json");
public static final MimeType APPLICATION_XML = parseMimeType("application/xml");
public static final MimeType APPLICATION_HTML_TEXT = parseMimeType("text/html");
private static Map<String, sybrix.easygsp2.routing.Route> routes = new LinkedHashMap<String, sybrix.easygsp2.routing.Route>();
private GroovyClassLoader groovyClassLoader;
private PropertiesFile propertiesFile;
private ServletContext context;
protected Serializer jsonSerializerInstance;
protected XmlSerializer xmlSerializerInstance;
protected List<Pattern> ignoredUrlPatterns;
private Set<String> classesWithApiAnnotation = new HashSet<String>();
private Map<String, String> methods = new HashMap<String, String>();
private boolean isServlet = false;
private AppListener appListener;
private Validator validator;
private EmailService emailService;
private AuthenticationService authenticationService;
private Map<String, Object> dynamicProperties = new HashMap<>();
public static EasyGsp2 instance;
public EasyGsp2(FilterConfig config) {
init(config.getServletContext(), config);
}
public EasyGsp2(ServletConfig config) {
init(config.getServletContext(), config);
}
public void init(ServletContext servletContext, Object config) {
try {
instance = this;
this.context = servletContext;
System.setProperty("easygsp.version", "@easygsp_version");
logger.info(
"\nEASYGSP_VERSION: " + System.getProperty("easygsp.version") +
"\nJRE_HOME: " + System.getProperty("java.home") +
"\nJAVA_VERSION: " + System.getProperty("java.version") +
"\nGROOVY_VERSION: " + GroovySystem.getVersion() +
"\n"
);
propertiesFile = new PropertiesFile("classPath:easygsp.properties");
servletContext.setAttribute(PropertiesFile.KEY, propertiesFile);
if (config instanceof ServletConfig) {
isServlet = true;
}
// delete tmp dir
File folder = new File(propertiesFile.getString("easygsp.tmp.dir", System.getProperty("java.io.tmpdir")));
if (folder.exists()) {
FileUtils.cleanDirectory(folder);
} else {
folder.mkdirs();
}
CompilerConfiguration configuration = new CompilerConfiguration();
configuration.setTargetDirectory(getCompiledClassesDir());
//configuration.setRecompileGroovySource(propertiesFile.getString("mode", "dev1").equalsIgnoreCase("dev"));
groovyClassLoader = new GroovyClassLoader(this.getClass().getClassLoader(), configuration);
//groovyClassLoader.addURL(getSourcePath(propertiesFile));
ignoredUrlPatterns = new ArrayList<Pattern>();
ThreadBag.set(new ThreadVariables(this.context, null, null, routes, null, null, groovyClassLoader));
if (propertiesFile.getString("authentication.service") != null) {
Class clsAuth = Class.forName(propertiesFile.getString("authentication.service"));
authenticationService = (AuthenticationService) clsAuth.newInstance();
} else {
logger.debug("No authentication.service value found");
}
if (propertiesFile.getString("email.service") != null) {
emailService = (EmailService) Class.forName(propertiesFile.getString("email.service")).newInstance();
context.setAttribute("emailService", emailService);
} else {
logger.debug("No email.service value found");
}
loadDefaultRoutes();//1
loadApiMethods(propertiesFile);//2
loadUnannotatedClasses(propertiesFile, "controllers.package"); //3 order matters
loadUnannotatedClasses(propertiesFile, "api.controllers.package"); //3 order matters
loadSerializers(propertiesFile);
loadPropertiesIntoContext(servletContext, propertiesFile);
loadJwtValues(propertiesFile);
String appListenerClass = null;
if (config instanceof ServletConfig) {
appListenerClass = ((ServletConfig) config).getInitParameter("appListener");
} else if (config instanceof FilterConfig) {
appListenerClass = ((FilterConfig) config).getInitParameter("appListener");
}
// load DB String queries
ThreadBag.set(new ThreadVariables(servletContext));
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
validator = factory.getValidator();
if (appListenerClass != null) {
Class cls = Class.forName(appListenerClass, false, groovyClassLoader);
//appListener = (AppListener) cls.newInstance();
appListener = (AppListener) ClassFactory.create(cls, this);
appListener.onApplicationStart(servletContext);
}
} catch (Throwable e) {
logger.error("error occurred easygsp init()", e);
} finally {
ThreadBag.remove();
}
}
private void loadDefaultRoutes() {
if (propertiesFile.getBoolean("jwt.controller.enabled", false)) {
Routes.add("POST", propertiesFile.getString("jwt.token.url", "/api/token"), JwtController.class, "generateToken", new Class[]{HttpServletRequest.class, HttpServletResponse.class}, null, new String[]{MediaType.JSON}, new String[]{MediaType.JSON}, false);
Routes.add("POST", propertiesFile.getString("jwt.tokenMobile.url", "/api/tokenMobile"), JwtController.class, "generateTokenFromPhone", new Class[]{HttpServletRequest.class, HttpServletResponse.class}, null, new String[]{MediaType.JSON}, new String[]{MediaType.JSON}, false);
Routes.add("POST", "/client", JwtController.class, "createClient", new Class[]{CreateClientRequest.class, HttpServletRequest.class}, new String[]{}, new String[]{MediaType.JSON}, new String[]{MediaType.JSON}, true);
}
}
private void loadJwtValues(PropertiesFile propertiesFile) {
JwtUtil jwtUtil = new JwtUtil(propertiesFile.getString("jwt.alg", "HS256"));
String key = propertiesFile.getString("jwt.key");
if (key == null) {
logger.info("no jwt key found. generating key....");
key = jwtUtil.generateKey();
logger.info("generated jwt key, HS256: " + key);
}
jwtUtil.loadKey(key);
JwtUtil.instance = jwtUtil;
}
protected AppListener getAppListener() {
return appListener;
}
public String getCompiledClassesDir() {
try {
File f = new File(this.getClass().getClassLoader().getResource("./../../WEB-INF/classes").toURI());
logger.info("compiled classed dir: " + f.getAbsolutePath());
return f.getAbsolutePath();
} catch (Exception e) {
throw new RuntimeException("failed on getCompiledClassesDir()", e);
}
}
private URL getSourcePath(PropertiesFile propertiesFile) {
String dir = propertiesFile.getProperty("source.dir", null);
URL url = null;
try {
if (dir != null) {
url = new File(dir).toURI().toURL();
} else {
url = this.getClass().getClassLoader().getResource("./../../");
}
logger.info("source path: " + url.getFile());
return url;
} catch (MalformedURLException e) {
throw new RuntimeException("Failed to determine source directory. " + e.getMessage(), e);
}
}
public boolean doRequest(final EasyGspServletRequest httpServletRequest, final HttpServletResponse httpServletResponse) throws ServletException {
String uri = null;
String contextPath = httpServletRequest.getServletContext().getContextPath();
if (contextPath.length() > 1) {
uri = httpServletRequest.getRequestURI().substring(contextPath.length());
} else {
uri = httpServletRequest.getRequestURI();
}
logger.debug("processing web request, method: " + httpServletRequest.getMethod() + ", uri: " + uri);
Boolean continueFilterChain = false;
List<MimeType> acceptHeaderMimeTypes;
try {
acceptHeaderMimeTypes = parseAcceptHeader(httpServletRequest.getHeader("Accept"));
for (Pattern s : ignoredUrlPatterns) {
if (s.matcher(uri).matches()) {
logger.debug("url: " + uri + " found on ignore list");
return true;
}
}
// default it to null, if you set it we'll know
httpServletResponse.setContentType(null);
final boolean isMultiPart = isMultiPart(httpServletRequest.getContentType());
final List<FileItem> uploads = FileUpload.parseFileUploads(httpServletRequest, propertiesFile);
ThreadBag.set(new ThreadVariables(this.context, httpServletRequest, httpServletResponse, routes, null, null, groovyClassLoader));
logger.debug("searching for matching route for uri: " + uri);
final sybrix.easygsp2.routing.Route route = findRoute(uri, httpServletRequest.getMethod());
if (route == null) {
if (httpServletRequest.getMethod().equalsIgnoreCase("GET")) {
logger.warn("remember- unannotated get() requires an Id parameter {<\\d+$>id}, index() does not");
}
throw new NoMatchingRouteException("No route found for: " + uri);
}
String extension = StringUtil.extractFileExtension(uri);
Closure closure = new Closure(this) {
Class controllerClass = null;
boolean isApiController = false;
public Object call() {
MimeType returnContentType = null;
try {
checkUserAuthorization(route, httpServletRequest);
appListener.onRequestStart(httpServletRequest);
String contentType = httpServletRequest.getContentType();
int contentLength = httpServletRequest.getContentLength();
Method m = null;
controllerClass = route.getControllerClass();
m = route.getControllerMethod();
GroovyObject controller = ClassFactory.create(controllerClass, getOwner());
List<String> s = lookupParameterNames(m, groovyClassLoader);
Parameter[] parameters = m.getParameters();
logger.debug("invoking method " + controllerClass.getName() + "." + m.getName() + "(), parameters: " + extractParameterTypes(parameters));
logger.debug("parameter names: " + s);
Enumeration<String> names = httpServletRequest.getHeaderNames();
while (names.hasMoreElements()) {
String header = names.nextElement();
logger.debug("header name/value: " + header + ":" + httpServletRequest.getHeader(header));
}
Object[] params = new Object[parameters.length];
int i = 0;
for (Parameter p : parameters) {
String parameterName = p.getName();
if (s.size() >= parameters.length) {
parameterName = s.get(i);
}
Class clazz = p.getType();
Type genericType = extractGenericType(p);
if (clazz == javax.servlet.http.HttpServletRequest.class || parameterName.equalsIgnoreCase("request")) {
params[i] = httpServletRequest;
} else if (clazz == HttpServletResponse.class || parameterName.equalsIgnoreCase("response")) {
params[i] = httpServletResponse;
} else if ((clazz == List.class && genericType == FileItem.class) || parameterName.equalsIgnoreCase("uploads") && isMultiPart) {
params[i] = uploads;
} else if ((clazz == FileItem.class) && isMultiPart) {
params[i] = getFileItemByName(uploads, parameterName);
} else if (clazz == ServletContext.class || parameterName.equalsIgnoreCase("app")) {
params[i] = httpServletRequest.getServletContext();
} else if (clazz == RequestContext.class || parameterName.equalsIgnoreCase("context")) {
RequestContext requestContext = new RequestContext();
requestContext.setApp(httpServletRequest.getServletContext());
requestContext.setProperties(propertiesFile);
requestContext.setUserPrincipal(httpServletRequest.getUserPrincipal());
requestContext.setRequest(httpServletRequest);
requestContext.setResponse(httpServletResponse);
params[i] = requestContext;
} else if (clazz == PropertiesFile.class || parameterName.equalsIgnoreCase("propertiesFile")) {
params[i] = propertiesFile;
} else if (clazz == UserPrincipal.class || clazz == Principal.class) {
params[i] = httpServletRequest.getUserPrincipal();
} else {
String valFromRequestParameter = null;
if (route.getParameters().size() > 0) {
UrlParameter urlParameter = route.getParameters().get(parameterName);
if (urlParameter == null && m.getName().equalsIgnoreCase("get")) {
logger.debug("unannotated method, did not find default parameter named \"id\", trying \"" + parameterName + "\" instead");
urlParameter = route.getParameters().get("id");
if (urlParameter == null) {
throw new BadRequestException("parameter name 'id' required. parameter name: " + parameterName);
}
}
if (urlParameter.getValue() != null) {
valFromRequestParameter = urlParameter.getValue();
} else if (valFromRequestParameter == null) {
valFromRequestParameter = httpServletRequest.getParameter(parameterName);
}
} else {
valFromRequestParameter = httpServletRequest.getParameter(parameterName);
}
if (valFromRequestParameter != null) {
params[i] = castToType(valFromRequestParameter, clazz);
} else if (contentLength > 0 && isJson(contentType)) {
Object obj = null;
if (clazz.getTypeName().equals("java.lang.Object")) {
obj = jsonSerializerInstance.parse(httpServletRequest.getInputStream(), contentLength);
} else {
obj = jsonSerializerInstance.parse(httpServletRequest.getInputStream(), contentLength, clazz, genericType);
}
params[i] = obj;
} else if (contentLength > 0 && isXML(contentType)) {
Object obj = null;
if (clazz.getTypeName().equals("java.lang.Object")) {
obj = xmlSerializerInstance.fromXml(httpServletRequest.getInputStream(), contentLength);
} else {
obj = xmlSerializerInstance.fromXml(httpServletRequest.getInputStream(), contentLength, clazz);
}
params[i] = obj;
} else { //if (contentType != null && contentLength > 0 && isFormUrlEncoded(contentType) ||) {
Object obj = clazz.newInstance();
populateBean(obj, httpServletRequest);
params[i] = obj;
}
}
i++;
}
//checkUserAuthorization(route, httpServletRequest);
isApiController = isApiController(controllerClass);
returnContentType = getContentReturnType(isApiController, httpServletResponse, acceptHeaderMimeTypes, route, extension);
List errors = validateParameters(params);
if (errors.size() > 0) {
throw new BadRequestException(errors);
}
Object _controllerResponse = invokeControllerAction(controller, m, params, route);
ControllerResponse controllerResponse = ControllerResponse.parse(_controllerResponse);
if (controllerResponse.forwarded()) {
httpServletRequest.getRequestDispatcher(controllerResponse.getUrl()).forward(httpServletRequest, httpServletResponse);
return false; // return now, don't continue filter chain.
}
if (controllerResponse.redirected()) {
httpServletResponse.sendRedirect(controllerResponse.getUrl());
return false; // return now, don't continue filter chain.
}
if (controllerResponse.getHttpCode() != null) {
httpServletResponse.setStatus(controllerResponse.getHttpCode());
}
logger.debug("controllers returned: " + _controllerResponse);
Object redirectIssued = httpServletRequest.getAttribute("__redirect__");
if (Boolean.TRUE.equals(redirectIssued)) {
return false;
}
logger.debug("return content type: " + returnContentType.toString());
if (returnContentType.getBaseType().equals(APPLICATION_HTML_TEXT.getBaseType())) {
logger.debug("processing view " + _controllerResponse);
ThreadBag.get().getTemplateInfo().setErrorOccurred(false);
return processControllerResponse(controllerResponse, httpServletResponse, httpServletRequest);
} else {
if (returnContentType.getBaseType().equals(APPLICATION_JSON.getBaseType())) {
//httpServletResponse.flushBuffer();
if (controllerResponse.getIsEntity() && controllerResponse.getEntity() != null) {
logger.debug("sending json response to client");
jsonSerializerInstance.write(controllerResponse.getEntity(), httpServletResponse.getOutputStream());
//httpServletResponse.flushBuffer();
} else {
logger.debug("controllers method returned null object, that's a 200 OK");
}
}
}
return false;
} catch (UnauthorizedException e) {
//if (e.getMessage().equalsIgnoreCase("EMAIL_NOT_VALIDATED")) {
processException(e, true, returnContentType, httpServletRequest, httpServletResponse);
//}
return false;
} catch (HttpException e) {
logger.debug(e.getMessage(), e);
processException(e, isApiController, returnContentType, httpServletRequest, httpServletResponse);
return false;
} catch (java.lang.IllegalArgumentException e) {
logger.error("controllers", e);
// } catch (NoMatchingRouteException | NoMatchingAcceptHeaderException | UnauthorizedException e) {
// sendError(e.getStatus(), new CustomServletBinding(httpServletRequest.getServletContext(), httpServletRequest, httpServletResponse), e.getCause().getCause());
// return false;
} catch (Exception e) {
logger.error(e.getMessage(), e);
processException(new HttpException(e, HttpServletResponse.SC_INTERNAL_SERVER_ERROR), isApiController, returnContentType, httpServletRequest, httpServletResponse);
return false;
}
return null;
}
};
continueFilterChain = (Boolean) GroovyCategorySupport.use(CustomServletCategory.class, closure);
} catch (NoMatchingRouteException e) {
if (isContentTypeAccepted(httpServletRequest, APPLICATION_JSON)) {
processException(e, true, APPLICATION_JSON, httpServletRequest, httpServletResponse);
} else {
sendError(e.getStatus(), new CustomServletBinding(this.context, httpServletRequest, httpServletResponse), e);
}
continueFilterChain = false;
} catch (InvokeControllerActionException e) {
sendError(500, new CustomServletBinding(this.context, httpServletRequest, httpServletResponse), e.getCause().getCause());
//throw new ServletException(e.getCause());
} catch (Exception e) {
logger.error(e.getMessage(), e);
sendError(500, new CustomServletBinding(this.context, httpServletRequest, httpServletResponse), e.getCause().getCause());
//throw new ServletException(e);
} finally {
try {
if (appListener != null) {
appListener.onRequestEnd(httpServletRequest);
}
} catch (Throwable e) {
e.printStackTrace();
}
ThreadBag.remove();
}
return continueFilterChain;
}
public MimeType getContentReturnType(Boolean apiRequest, HttpServletResponse httpServletResponse, List<MimeType> acceptHeaderMimeTypes, Route route, String extension) throws MimeTypeParseException {
if (httpServletResponse.getContentType() == null) {
MimeType m = determineMimeTypeToReturn(apiRequest, acceptHeaderMimeTypes, route, extension);
httpServletResponse.setContentType(m.toString());
return m;
} else {
return new MimeType(httpServletResponse.getContentType());
}
}
public void processException(HttpException e, boolean apiRequest, MimeType returnContentType, HttpServletRequest httpServletRequest, HttpServletResponse httpServletResponse) {
try {
httpServletResponse.setStatus(e.getStatus());
if (returnContentType.getBaseType().equals(APPLICATION_XML.getBaseType())) {
//msg = xmlSerializerInstance.toString(e.getConstraintErrors());
logger.error("IMPLEMENT XML toString on Serializer");
} else if (returnContentType.getBaseType().equals(APPLICATION_JSON.getBaseType()) && apiRequest) {
String msg = "";
if (e.getStatus() == 500) {
ApiError apiError = new ApiError("Server error occurred. Try again.");
apiError.setCode(e.getStatus());
if (e instanceof BadRequestException) {
apiError.setMessages(((BadRequestException) e).getConstraintErrors());
}
msg = jsonSerializerInstance.toString(apiError);
} else {
ApiError apiError = new ApiError(e.getMessage());
if (e.getErrorCode() != null) {
apiError.setCode(e.getErrorCode());
} else {
apiError.setCode(e.getStatus());
}
if (e instanceof BadRequestException) {
apiError.setMessages(((BadRequestException) e).getConstraintErrors());
}
msg = jsonSerializerInstance.toString(apiError);
}
httpServletResponse.getOutputStream().write(msg.getBytes());
} else if (returnContentType.getBaseType().equals(APPLICATION_HTML_TEXT.getBaseType())) {
sendError(e.getStatus(), new CustomServletBinding(httpServletRequest.getServletContext(), httpServletRequest, httpServletResponse), extractException(e));
}
} catch (Exception x) {
logger.debug("error occurred writing back to client on bad request", e);
}
}
private Throwable extractException(HttpException e) {
if (e.getCause() == null)
return e;
else {
return e.getCause().getCause();
}
}
private void checkUserAuthorization(Route route, EasyGspServletRequest httpServletRequest) throws UnauthorizedException {
try {
if (route.isSecure()) {
UserPrincipal userPrincipal = (UserPrincipal) httpServletRequest.getUserPrincipal();
if (userPrincipal.getId() == null) {
throw new UnauthorizedException();
}
if (route.getRoles().size() == 0) {
// only authentication is required when route.getRoles.size ==0
return;
}
// check for role
for (String role : route.getRoles()) {
if (httpServletRequest.isUserInRole(role)) {
return;
}
}
throw new UnauthorizedException();
}
} catch (ExpiredJwtException | NullPointerException e) {
throw new UnauthorizedException();
}
}
public static Cookie getCookie(String cookieName, HttpServletRequest request) {
Cookie c[] = request.getCookies();
if (c != null) {
for (int x = 0; x < c.length; x++) {
if (c[x].getName().equals(cookieName)) {
return c[x];
}
}
}
return null;
}
private List<ValidationMessage> validateParameters(Object[] params) {
List constraintViolations = new ArrayList();
for (Object o : params) {
if (o == null || o instanceof ServletContext || o instanceof FileItem || o instanceof ServletRequest || o instanceof ServletResponse) {
continue;
}
Set _constraintViolations = validator.validate(o);
for (Object c : _constraintViolations) {
ConstraintViolation constraintViolation = ((ConstraintViolation) c);
ValidationMessage vm = new ValidationMessage(constraintViolation.getPropertyPath().toString(), constraintViolation.getMessage());
constraintViolations.add(vm);
}
}
return constraintViolations;
}
private MimeType determineMimeTypeToReturn(Boolean apiRequest, List<MimeType> accepts, Route route, String extension) throws MimeTypeParseException {
if (accepts == null) {
accepts = new ArrayList<MimeType>();
}
if (accepts.size() == 0) {
if (apiRequest == true) {
return MimeTypes.getMimeTypes().get(".json");
} else {
return MimeTypes.getMimeTypes().get(".html");
}
}
if (!StringUtil.isEmpty(extension) && route.getProducesMimeType().size() == 0) {
if (MimeTypes.getMimeTypes().containsKey(extension)) {
return MimeTypes.getMimeTypes().get(extension);
}
}
if (apiRequest) {
route.setProduces(new String[]{propertiesFile.getString("default.response.contentType", MediaType.JSON)});
}
for (MimeType accept : accepts) {
MimeType m = isContentTypeAccepted(accept, route.getProducesMimeType());
if (m != null) {
return m;
}
}
throw new UnsupportedContentType("");
// check method
// check class
// check default return type
}
private MimeType calculateContentType(ControllerResponse controllerResponse, sybrix.easygsp2.routing.Route route, HttpServletResponse response, HttpServletRequest request, Boolean isApiCall) throws MimeTypeParseException {
// if there's only one, the use it
if (route.getProduces().length == 1) {
return new MimeType(route.getProduces()[0]);
} else if (route.getProduces().length == 0 && controllerResponse.getIsString()) {
return APPLICATION_HTML_TEXT;
}
// if you set it manually use it
if (response.getContentType() != null && response.getContentType().length() > 0) {
return new MimeType(response.getContentType());
}
MimeType defaultMimeType = null;
if (isApiCall) {
defaultMimeType = new MimeType(propertiesFile.getString("api.default.response.contentType", "application/json"));
} else {
defaultMimeType = new MimeType(propertiesFile.getString("default.response.contentType", "text/html"));
}
// if you don't set, figure it out based on what you returned from the method
if (route.getProduces().length == 0) {
if (controllerResponse.getIsJsonSluper()) {
return APPLICATION_JSON;
} else if (controllerResponse.getIsXMLSlurper()) {
return APPLICATION_XML;
} else {
if (defaultMimeType.getBaseType().equals(APPLICATION_JSON.getBaseType())) {
return APPLICATION_JSON;
} else if (defaultMimeType.getBaseType().equals(APPLICATION_XML.getBaseType())) {
return APPLICATION_XML;
} else {
return defaultMimeType;
}
}
}
return defaultMimeType;
}
private List<MimeType> parseAcceptHeader(String acceptHeader) {
List<MimeType> accepts = new ArrayList();
String header = null;
if (acceptHeader != null) {
try {
String[] parts = acceptHeader.split(",");
for (String s : parts) {
header = s;
accepts.add(new MimeType(header));
}
} catch (MimeTypeParseException e) {
logger.debug("MimeTypeParseException, " + e.getMessage() + ", acceptHeader Param:" + acceptHeader + ", bad header: " + header);
}
}
return accepts;
}
// private boolean isContentTypeAccepted(){
//
// String accept = request.getHeader("Accept");
// String[] acceptsHeader = {};
//
// if (accept != null) {
// acceptsHeader = accept.split(",");
// }
//
// // no accepts header
// if (acceptsHeader == null) {
// acceptsHeader = route.getProduces().length == 0 ? defaultContentType.split(",") : route.getProduces()[0].split(",");
// }
//
// String[] returns = route.getProduces();
// String[] returnContentType = null;
// if (returns.length == 0) {
// returnContentType = defaultContentType.split(",");
// }
//
//
// MimeType mimeType = findMimeTypeMatch(acceptsHeader, returnContentType);
//
// if (mimeType != null) {
// response.setContentType(mimeType.toString());
// return mimeType.toString();
// } else {
// logger.info("unable to determine response content type");
// return null;
// }
//
// }
private MimeType isContentTypeAccepted(MimeType sought, List<MimeType> listToSearch) {
if (listToSearch.size() == 0) {
logger.debug("no \"produces\" in @Api annotation, letting it through");
return null;
}
for (MimeType m : listToSearch) {
if (sought.match(m) || m.getPrimaryType().equals("*") || sought.getPrimaryType().equals("*")) {
return m;
}
}
if (sought.getPrimaryType().equals("*") && listToSearch.size() == 0) {
return sought;
}
return null;
}
private boolean isContentTypeAccepted(HttpServletRequest request, MimeType soughtMimeType) {
try {
if (request.getHeader("Accept") == null) {
return true;
}
for (String r : request.getHeader("Accept").split(",")) {
MimeType mimeType = new MimeType(r);
if (soughtMimeType.match(mimeType) || mimeType.getPrimaryType().equals("*")) {
return true;
}
}
return false;
} catch (Exception e) {
logger.debug(e.getMessage(), e);
return false;
}
}
private MimeType findMimeTypeMatch(String[] acceptsHeader, String[] returnContentType) {
try {
if (returnContentType == null) {
return null;
}
for (String r : returnContentType) {
MimeType returnMime = new MimeType(r);
for (String a : acceptsHeader) {
if (returnMime.match(a)) {
return returnMime;
}
}
}
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
private boolean compare(String s1, String s2) {
return s1.trim().toLowerCase().equals(s2.trim().toLowerCase());
}
private FileItem getFileItemByName(List<FileItem> uploads, String parameterName) {
for (FileItem i : uploads) {
if (i.getFieldName().equals(parameterName)) {
return i;
}
}
return null;
}
private Type extractGenericType(Parameter p) {
try {
return ((ParameterizedTypeImpl) p.getParameterizedType()).getActualTypeArguments()[0];
} catch (Exception e) {
return null;
}
}
private boolean processControllerResponse(ControllerResponse controllerResponse, HttpServletResponse httpServletResponse, HttpServletRequest httpServletRequest) throws URISyntaxException, IOException, ServletException {
ThreadBag.get().setTemplateRequest(true);
Binding binding = ThreadBag.get().getBinding();
if (binding == null) {
binding = new CustomServletBinding(context, httpServletRequest, httpServletResponse);
}
if (controllerResponse.getIsString() && controllerResponse.getEntity().toString().endsWith(".jsp") && isServlet) {
RequestDispatcher rd = httpServletRequest.getServletContext().getRequestDispatcher("/indexs.jsp");
rd.forward(httpServletRequest, httpServletResponse);
} else if (controllerResponse.getIsString() && controllerResponse.getEntity().toString().endsWith(".jsp") && isServlet == false) {
RequestDispatcher rd = httpServletRequest.getServletContext().getRequestDispatcher("/indexs.jsp");
rd.forward(httpServletRequest, httpServletResponse);
return true;
} else if (controllerResponse.getIsString() && !controllerResponse.getEntity().toString().endsWith(".jsp")) {
URL f = Thread.currentThread().getContextClassLoader().getResource("./../../WEB-INF/views/" + controllerResponse.getViewTemplate());
if (f == null) {
throw new NoViewTemplateFound("View template '" + controllerResponse.toString() + "' not found!!!");
}
ThreadBag.get().setViewFolder(f.getPath());
File requestedViewFile = new File(f.toURI());
ThreadBag.get().setTemplateFilePath(requestedViewFile.getAbsolutePath());
ThreadBag.get().getTemplateInfo().setRequestUri(controllerResponse.toString());
ThreadBag.get().getTemplateInfo().setAppFolderClassPathLocation("./../../WEB-INF/views/");
ThreadBag.get().getTemplateInfo().setRequestFile(requestedViewFile);
try {
TemplateWriter templateWriter = new TemplateWriter(groovyClassLoader);
templateWriter.process(httpServletResponse, ThreadBag.get().getTemplateInfo(), binding);
return false;
} catch (HttpException e) {
logger.debug(e.getMessage(), e);
sendError(e.getStatus(), binding, e.getCause());
return false;
} catch (Throwable e) {
logger.debug(e.getMessage(), e);
sendError(500, binding, e.getCause());
return false;
}
}
return true;
}
protected void sendError(int httpStatusCode, Binding binding, Throwable e) {
try {
ThreadBag.get().getTemplateInfo().setErrorOccurred(true);
try {
// ThreadBag.get().getResponse().sendError(errorCode, e.getMessage());
} catch (IllegalStateException il) {
}
ThreadBag.get().getResponse().setStatus(httpStatusCode);
RequestError requestError = ThreadBag.get().getRequestError();
requestError.setErrorCode(httpStatusCode);
requestError.setScriptPath(ThreadBag.get().getTemplateFilePath());
requestError.setErrorMessage(e.getMessage() == null ? "" : e.getMessage());
requestError.setException(e, "", "");
StackTraceElement stackTraceElement = findErrorInStackTrace(binding, e);
String errorSource = highlightErrorSource(stackTraceElement);
requestError.setSource(errorSource.toString());
String path = ThreadBag.get().getTemplateFilePath();//.replace(appPath, "").replace(appPath2, "");
if (stackTraceElement != null) {
String lineNumberMessage = "Error occurred in <span class=\"path\">" + path + "</span> @ lineNumber: " + (stackTraceElement.getLineNumber());
requestError.setLineNumberMessage(lineNumberMessage);
requestError.setLineNumber(stackTraceElement.getLineNumber());
} else {
requestError.setLineNumberMessage("");
if (ThreadBag.get().isTemplateRequest()) {
String lineNumberMessage = "Error occurred in <span class=\"path\">" + path + "</span>";
requestError.setLineNumberMessage(lineNumberMessage);
}
requestError.setLineNumber(-1);
}
File errorFile = getErrorFileContents(httpStatusCode);
ThreadBag.get().getTemplateInfo().setRequestFile(errorFile);
binding.setVariable("exceptionName", e.getClass().getName());
binding.setVariable("requestError", requestError);
processErrorTemplateRequest(ThreadBag.get().getResponse(), ThreadBag.get().getTemplateInfo(), binding);
ThreadBag.get().getResponse().flushBuffer();
} catch (Throwable e1) {
logger.debug(e1.getMessage(), e1);
//sendError(errorCode, response, e, stackTraceElement, binding);
}
}
private String highlightErrorSource(StackTraceElement stackTraceElement) {
StringBuffer lineBuffer = new StringBuffer();
if (stackTraceElement != null) {
try {
File f = new File(ThreadBag.get().getTemplateFilePath());
BufferedReader br = new BufferedReader(new FileReader(f));
String line = null;
int lineCt = 1;
while ((line = br.readLine()) != null) {
if (lineCt >= stackTraceElement.getLineNumber() - 10 && (lineCt <= stackTraceElement.getLineNumber() + 10)) {
lineBuffer.append("<div class=\"sourceLine\"><div class=\"sourceLineNumber\">").append(lineCt).append("</div>");
lineBuffer.append("<div class=\"sourceCode\">");
lineBuffer.append(line.length() == 0 ? " " : StringUtil.htmlEncode(line).replaceAll(" ", " "));
lineBuffer.append("</div></div>");
}
lineCt++;
}
br.close();
} catch (Exception ex) {
logger.debug("Unable to parse source code to display on error page", ex);
}
}
return lineBuffer.toString();
}
private File getErrorFileContents(int errorCode) throws IOException {
String defaultTemplateExtension = propertiesFile.getString("default.template.extension", "gsp");
String tmpDir = propertiesFile.getString("easygsp.tmp.dir", System.getProperty("java.io.tmpdir"));
InputStream inputStream = Thread.currentThread().getContextClassLoader().getResourceAsStream("./errors/error" + errorCode + "." + defaultTemplateExtension);
File errorFile = new File(tmpDir + File.separator + "errors" + File.separator + "error" + errorCode + "." + defaultTemplateExtension);
// createFile if it doesn't exist
if (!errorFile.exists()) {
new File(tmpDir + File.separator + "errors").mkdir();
FileOutputStream outputStream = new FileOutputStream(errorFile);
byte[] buff = new byte[1024];
while (true) {
int len = inputStream.read(buff, 0, buff.length);
if (len < 0)
break;
outputStream.write(buff, 0, len);
}
outputStream.close();
}
return errorFile;
}
private void processErrorTemplateRequest(HttpServletResponse httpServletResponse, TemplateInfo templateInfo, Binding binding) {
try {
TemplateWriter templateWriter = new TemplateWriter(groovyClassLoader);
templateWriter.process(httpServletResponse, templateInfo, binding);
} catch (Exception e) {
e.printStackTrace();
}
}
private static StackTraceElement findErrorInStackTrace(Binding binding, Throwable e) {
if (ThreadBag.get().isTemplateRequest()) {
if (e.getStackTrace() != null && ThreadBag.get().getTemplateInfo() != null) {
for (StackTraceElement stackTraceElement : e.getStackTrace()) {
if (stackTraceElement.getFileName() != null) {
if (stackTraceElement.getFileName().startsWith(ThreadBag.get().getTemplateInfo().getUniqueTemplateScriptName())) {
return stackTraceElement;
}
}
}
}
} else {
// String appPath = RequestThreadInfo.get().getApplication().getAppPath();
// String currentFile = ThreadBag.get().getTemplateFilePath().substring(appPath.length() + 1);
//
// for (StackTraceElement stackTraceElement : e.getStackTrace()) {
// if (stackTraceElement.getFileName() != null) {
// if (stackTraceElement.getFileName().endsWith(currentFile)) {
// return stackTraceElement;
// }
// }
// }
}
return null;
}
private void loadPropertiesIntoContext(ServletContext app, PropertiesFile propertiesFile) {
for (String s : propertiesFile.stringPropertyNames()) {
app.setAttribute(s, propertiesFile.get(s));
if (s.matches("ignore\\.url\\.pattern\\.\\d+$")) {
ignoredUrlPatterns.add(Pattern.compile(propertiesFile.getString(s)));
}
}
}
private void loadUnannotatedClasses(PropertiesFile propertiesFile, String packageName) {
logger.debug("loading unannotated controllers classes");
String defaultPackageName = propertiesFile.getString(packageName);
Reflections reflections = new Reflections(defaultPackageName, new TypeElementsScanner(), new SubTypesScanner(false));
Set<String> types = reflections.getAllTypes();
for (String cls : types) {
if (classesWithApiAnnotation.contains(cls)) {
// continue;
}
if (cls.endsWith("Controller")) {
logger.debug("parsing class: " + cls);
StringBuffer sb = new StringBuffer();
String _api = cls.substring(defaultPackageName.length() + 1, cls.length() - "Controller".length());
for (String s : _api.split("\\.")) {
sb.append(s.substring(0, 1).toLowerCase());
sb.append(s.substring(1, s.length()));
sb.append("/");
}
String api = sb.substring(0, sb.length() - 1);
String pattern = "/" + api;
Class clazz = parseClassName(cls);
String[] classMethods = {"list", "index", "get", "put", "post", "delete"};
if (clazz != null) {
String mediaType[] = {propertiesFile.getString("default.response.contentType", MediaType.HTML)};
String[] accepts = mediaType;
String[] returns = determineDefaultReturnType(clazz);
boolean isApiController = isApiController(clazz);
Secured securedAnno = (Secured) clazz.getAnnotation(Secured.class);
String[] classRoles = securedAnno == null ? new String[]{} : securedAnno.value();
for (String classMethod : classMethods) {
String _pattern = pattern;
String _method = classMethod;
if (classMethod.equalsIgnoreCase("list")) {
if (isApiController) {
_method = "list";
} else {
_method = "index";
}
}
if (classMethod.equalsIgnoreCase("DELETE") || classMethod.equalsIgnoreCase("GET")) {
_pattern = pattern + "/{<\\d+$>id}";
}
String httpMethod = (classMethod.equalsIgnoreCase("list") || classMethod.equalsIgnoreCase("index")) ? "GET" : classMethod.toUpperCase();
if (!Routes.contains(_pattern, httpMethod)) {
MethodAndRole method = extractMethod(clazz, _method);
if (method.getSecure() == false) {
method.setSecure(securedAnno != null);
}
if (method.getMethod() != null) {
String[] roles = combine(method.getRoles(), classRoles);
Routes.add(method.getMethod(), _pattern, httpMethod, new Class[]{}, roles, accepts, returns, method.getSecure());
//route.setSecure();
}
}
}
}
} else {
logger.warn("class: " + cls + " doesn't end with 'Controller', skipping as controllers");
}
}
}
private boolean isApiController(Class clazz) {
String apiControllerPackage = propertiesFile.getString("api.controllers.package", "");
if (clazz.getPackage().getName().equals(apiControllerPackage)) {
return true;
}
return false;
}
private String[] determineDefaultReturnType(Class clazz) {
Content content = null;
try {
content = (Content) clazz.getAnnotation(Content.class);
if (content != null) {
String returnType = content.returns();
if (returnType != null) {
return new String[]{returnType};
}
}
} catch (Exception e) {
logger.debug("unable to get Content annotation form class " + clazz.getName());
}
String controllerPackage = propertiesFile.getString("controllers.package", "");
String apiControllerPackage = propertiesFile.getString("api.controllers.package", "");
if (clazz.getPackage().getName().equals(controllerPackage)) {
return new String[]{propertiesFile.getString("default.response.contentType", MediaType.JSON)};
} else if (clazz.getPackage().getName().equals(apiControllerPackage)) {
return new String[]{propertiesFile.getString("api.default.response.contentType", MediaType.HTML)};
}
return new String[]{};
}
private Class parseClassName(String cls) {
try {
return Class.forName(cls);
} catch (ClassNotFoundException e) {
return null;
}
}
private MethodAndRole extractMethod(Class cls, String methodName) {
MethodAndRole methodAndRole = new MethodAndRole();
try {
Method foundMethod = null;
for (Method m : cls.getDeclaredMethods()) {
if (m.getName().equals(methodName)) {
if (foundMethod == null) {
foundMethod = m;
} else {
logger.error("method " + methodName + "() found in class: " + cls.getName() + ", not allowed in when using routing by naming convention only. Use @api annotation instead.");
break;
}
}
}
if (foundMethod == null) {
return methodAndRole;
}
Method m = foundMethod;
methodAndRole.setMethod(m);
Annotation annotation = m.getDeclaredAnnotation(Secured.class);
if (annotation != null) {
methodAndRole.setSecure(true);
String[] roles = ((Secured) annotation).value();
methodAndRole.setRoles(roles);
}
} catch (Exception e) {
logger.debug(methodName.toLowerCase() + "() method not found in class " + cls.getName());
return methodAndRole;
}
return methodAndRole;
}
private void loadApiMethods(PropertiesFile propertiesFile) {
logger.debug("loading annotated controllers methods...");
Reflections reflections = new Reflections("", new MethodAnnotationsScanner(), new SubTypesScanner());
Set<Method> methods = reflections.getMethodsAnnotatedWith(Api.class);
for (Method m : methods) {
Api classAnno = extractClassAnnotation(m.getDeclaringClass().getDeclaredAnnotations());
classesWithApiAnnotation.add(m.getDeclaringClass().getName());
Api methodAnno = m.getDeclaredAnnotation(Api.class);
Secured securedClassAnno = (Secured) m.getDeclaringClass().getAnnotation(Secured.class);
String[] classRolesFromSecureAnnotation = securedClassAnno == null ? new String[]{} : securedClassAnno.value();
Secured securedMethodAnno = m.getDeclaredAnnotation(Secured.class);
String[] methodRolesFromSecureAnnotation = securedMethodAnno == null ? new String[]{} : securedMethodAnno.value();
boolean secured = (securedMethodAnno != null || securedClassAnno != null);
if (methodAnno.url().length > 0) {
logger.debug("loading class: " + m.getDeclaringClass().getName() + ", method: " + m.getName() + "(), url: " + methodAnno.url());
for (String classPattern : classAnno.url()) {
for (String _pattern : methodAnno.url()) {
String pattern = classPattern + _pattern;
String[] httpMethods = combine(methodAnno.method(), classAnno.method());
for (String httpMethod : httpMethods) {
if (httpMethod.equals("*")) {
httpMethods = sybrix.easygsp2.routing.Route.HTTP_METHODS;
break;
}
}
for (String httpMethod : httpMethods) {
String[] securedRoles = combine(classRolesFromSecureAnnotation, methodRolesFromSecureAnnotation);
String[] roles = combine(methodAnno.roles(), securedRoles, classAnno.roles());
String[] accepts = methodAnno.accepts() != null ? classAnno.accepts() : methodAnno.accepts();
String[] returns = methodAnno.contentType() != null ? classAnno.contentType() : methodAnno.contentType();
if (returns.length == 0) {
returns = determineDefaultReturnType(m.getDeclaringClass());
}
Routes.add(m, pattern, httpMethod, null, roles, accepts, returns, secured);
}
}
}
} else {
logger.info("no patterns found for method " + m);
}
}
}
private Api extractClassAnnotation(Annotation[] declaredAnnotations) {
for (Annotation declaredAnnotation : declaredAnnotations) {
if (declaredAnnotation instanceof Api) {
return (Api) declaredAnnotation;
}
}
return _dummy.class.getDeclaredAnnotation(Api.class);
}
private Set<String> toList(String[] methods) {
Set<String> l = new HashSet<String>();
if (methods != null) {
for (String m : methods) {
l.add(m);
}
}
return l;
}
private void loadSerializers(PropertiesFile propertiesFile) {
String jsonSerializer = null;
String xmlSerializer = null;
try {
jsonSerializer = propertiesFile.getString("json.serializer.class", JSON_SERIALIZER_CLASS);
logger.debug("JsonSerializer: " + jsonSerializer);
jsonSerializerInstance = (Serializer) Class.forName(jsonSerializer, false, groovyClassLoader).newInstance();
} catch (Exception e) {
if (JSON_SERIALIZER_CLASS.equalsIgnoreCase(jsonSerializer)) {
logger.error("Unable to instantiate default json serializer: " + JSON_SERIALIZER_CLASS);
} else {
logger.warn("error occurred instantiating jsonSerializer: " + jsonSerializer + ", attempting to use default: " + JSON_SERIALIZER_CLASS);
try {
jsonSerializerInstance = (Serializer) Class.forName(jsonSerializer, false, groovyClassLoader).newInstance();
} catch (Exception e1) {
logger.error("Unable to instantiate default json serializer: " + JSON_SERIALIZER_CLASS);
}
}
}
try {
xmlSerializer = propertiesFile.getString("xml.serializer.class", XML_SERIALIZER_CLASS);
logger.debug("XmlSerializer: " + xmlSerializer);
xmlSerializerInstance = (XmlSerializer) Class.forName(xmlSerializer, false, groovyClassLoader).newInstance();
} catch (Exception e) {
logger.warn("error occurred instantiating xmlSerializer: " + xmlSerializer + ", attempting to use default: " + XML_SERIALIZER_CLASS);
try {
xmlSerializerInstance = (XmlSerializer) Class.forName(xmlSerializer, false, groovyClassLoader).newInstance();
} catch (Exception e1) {
logger.error("Unable to instantiate default xml serializer: " + XML_SERIALIZER_CLASS);
}
}
}
private Object invokeControllerAction(GroovyObject controller, Method m, Object[] params, sybrix.easygsp2.routing.Route route) throws HttpException, InvokeControllerActionException {
try {
return m.invoke(controller, params);
} catch (Exception e) {
if (e.getCause() instanceof HttpException) {
throw (HttpException) e.getCause();
} else {
if (route.isDuplicate()) {
logger.error("url pattern: " + route.getPath() + " exists on multiple methods.");
}
logger.error(e.getMessage(), e);
throw new InvokeControllerActionException("unable to invoke method " + m.getDeclaringClass().getName() + "." + m.getName() + extractParameterTypes(m.getParameters()) + ", attempted: " + m.getName() + extractParameterTypes(params), e);
}
}
}
public Object populateBean(Object obj, HttpServletRequest request) {
try {
Object value = null;
String property = null;
BeanInfo sourceInfo = Introspector.getBeanInfo(obj.getClass());
PropertyDescriptor[] sourceDescriptors = sourceInfo.getPropertyDescriptors();
for (int x = 0; x < sourceDescriptors.length; x++) {
try {
if (sourceDescriptors[x].getReadMethod() != null && sourceDescriptors[x].getWriteMethod() != null) {
property = sourceDescriptors[x].getName();
Class params[] = sourceDescriptors[x].getWriteMethod().getParameterTypes();
String val = request.getParameter(property);
if (val != null) {
value = castToType(val, Class.forName(params[0].getName()));
if (obj instanceof GroovyObject) {
MetaClass metaClass = InvokerHelper.getMetaClass(obj);
metaClass.setProperty(obj, sourceDescriptors[x].getName(), value);
} else {
sourceDescriptors[x].getWriteMethod().invoke(obj, new Object[]{value});
}
}
}
} catch (Exception e) {
logger.warn("BeanUtil.populate failed. method=" + property + ", value=" + value, e);
}
}
} catch (Exception e) {
logger.error("Error occurred populating object from request", e);
}
return obj;
}
private Object castToType(String valFromRequestParameter, Class clazz) {
try {
if (clazz == Object.class) {
return valFromRequestParameter;
} else if (clazz.getName().equals("char")) {
return new Character(valFromRequestParameter.toCharArray()[0]);
} else if (clazz.getName().equals("int")) {
return new Integer(valFromRequestParameter);
} else if (clazz.getName().equals("long") || clazz.getName().equals("java.lang.Long")) {
return new Long(valFromRequestParameter);
} else if (clazz.getName().equals("double")) {
return new Double(valFromRequestParameter);
} else if (clazz.getName().equals("float")) {
return new Float(valFromRequestParameter);
} else if (clazz.getName().equals("short")) {
return new Short(valFromRequestParameter);
} else if (clazz.getName().equals("boolean")) {
return new Boolean(valFromRequestParameter);
}
Constructor c = clazz.getConstructor(String.class);
return c.newInstance(valFromRequestParameter);
} catch (NoSuchMethodException e) {
e.printStackTrace();
} catch (InvocationTargetException e) {
e.printStackTrace();
} catch (InstantiationException e) {
e.printStackTrace();
} catch (IllegalAccessException e) {
e.printStackTrace();
}
return null;
}
private String extractParameterTypes(Parameter[] parameters) {
StringBuffer stringBuffer = new StringBuffer();
stringBuffer.append("(");
for (Parameter p : parameters) {
stringBuffer.append(p.getType().getName()).append(",");
}
if (stringBuffer.length() > 1) {
stringBuffer.setLength(stringBuffer.length() - 1);
}
stringBuffer.append(")");
return stringBuffer.toString();
}
private String extractParameterTypes(Object[] parameters) {
StringBuffer stringBuffer = new StringBuffer();
stringBuffer.append("(");
for (Object p : parameters) {
if (p == null) {
stringBuffer.append("null").append(",");
} else {
stringBuffer.append(p.getClass().getName()).append("=").append(p).append(",");
}
}
if (stringBuffer.length() > 1) {
stringBuffer.setLength(stringBuffer.length() - 1);
}
stringBuffer.append(")");
return stringBuffer.toString();
}
private void loadRoutes() throws IOException, URISyntaxException, ClassNotFoundException {
final Class c = groovyClassLoader.parseClass(new File(this.getClass().getClassLoader().getResource("./routes.groovy").toURI()));
Closure closure = new Closure(groovyClassLoader) {
public Object call() {
try {
Binding binding = new Binding();
binding.setVariable("rroutes", routes);
binding.setVariable("groovyClassLoader", groovyClassLoader);
Script routesScript = InvokerHelper.createScript(c, binding);
routesScript.invokeMethod("run", new Object[]{});
} catch (Exception e) {
logger.error("error in routes.groovy", e);
}
return null;
}
};
GroovyCategorySupport.use(RoutingCategory.class, closure);
}
public static sybrix.easygsp2.routing.Route findRoute(String uri, String method) {
for (sybrix.easygsp2.routing.Route r : routes.values()) {
if (r.matches(uri, method)) {
logger.debug("matching route found! " + r.toString());
return r;
}
}
logger.debug("no matching route found for " + uri);
return null;
}
private static boolean isJson(String contentType) {
return contentType == null ? false : contentType.contains(APPLICATION_JSON.getBaseType());
}
private static boolean isXML(String contentType) {
return contentType == null ? false : contentType.contains(APPLICATION_XML.getBaseType());
}
private static boolean isFormUrlEncoded(String contentType) {
return contentType.contains("application/x-www-form-urlencoded");
}
private static boolean isMultiPart(String contentType) {
if (contentType == null)
return false;
return contentType.startsWith("multipart/");
}
private String[] extractControllerAndActionName(String contextName, String uri) {
String[] parts = uri.split("/");
StringBuffer controller = new StringBuffer();
String action = "index";
if (parts.length == 0) {
controller.append("Default");
} else {
if (contextName.equals(parts[0])) {
controller.append(parts[1]);
if (parts.length > 2) {
action = parts[2];
}
} else {
controller.append(parts[0]);
if (parts.length > 1) {
action = parts[1];
}
}
}
controller.append("Controller");
controller.replace(0, 1, String.valueOf(controller.charAt(0)).toUpperCase());
return new String[]{controller.toString(), action};
}
//taken from playframework
public List<String> lookupParameterNames(Method method, ClassLoader classLoader) {
try {
List<String> parameters = new ArrayList<String>();
ClassPool classPool = new ClassPool();
classPool.appendSystemPath();
classPool.appendClassPath(new LoaderClassPath(this.getClass().getClassLoader()));
classPool.appendClassPath("/Users/dsmith/Temp/groovy");
CtClass ctClass = classPool.get(method.getDeclaringClass().getName());
CtClass[] cc = new CtClass[method.getParameterTypes().length];
for (int i = 0; i < method.getParameterTypes().length; i++) {
cc[i] = classPool.get(method.getParameterTypes()[i].getName());
}
CtMethod ctMethod = ctClass.getDeclaredMethod(method.getName(), cc);
// Signatures names
CodeAttribute codeAttribute = (CodeAttribute) ctMethod.getMethodInfo().getAttribute("Code");
if (codeAttribute != null) {
LocalVariableAttribute localVariableAttribute = (LocalVariableAttribute) codeAttribute.getAttribute("LocalVariableTable");
if (localVariableAttribute != null && localVariableAttribute.tableLength() >= ctMethod.getParameterTypes().length) {
for (int i = 0; i < localVariableAttribute.tableLength(); i++) {
String name = localVariableAttribute.getConstPool().getUtf8Info(localVariableAttribute.nameIndex(i));
if (!name.equals("this") && !parameters.contains(name)) {
parameters.add(name);
}
}
}
}
return parameters;
} catch (Exception e) {
throw new RuntimeException(e);
}
}
public static File toFile(String path) throws URISyntaxException {
URL f = Thread.currentThread().getContextClassLoader().getResource(path);
return new File(f.toURI());
}
@Api(url = "", method = "", roles = "")
private static class _dummy {
}
private static MimeType parseMimeType(String s) {
try {
return new MimeType(s);
} catch (MimeTypeParseException e) {
return null;
}
}
public Validator getValidator() {
return validator;
}
public void setValidator(Validator validator) {
this.validator = validator;
}
public PropertiesFile getPropertiesFile() {
return propertiesFile;
}
public EmailService getEmailService() {
return emailService;
}
public AuthenticationService getAuthenticationService() {
return authenticationService;
}
public Map<String, Object> getDynamicProperties() {
return dynamicProperties;
}
}
<file_sep>rootProject.name = 'easygsp2'
<file_sep>package sybrix.easygsp2.framework;
import groovy.lang.Binding;
import groovy.lang.GroovyClassLoader;
import groovy.sql.Sql;
import io.jsonwebtoken.Claims;
import io.jsonwebtoken.Jws;
import sybrix.easygsp2.routing.Route;
import sybrix.easygsp2.security.JwtUtil;
import sybrix.easygsp2.security.UserPrincipal;
import sybrix.easygsp2.templates.RequestError;
import sybrix.easygsp2.templates.TemplateInfo;
import javax.servlet.ServletContext;
import javax.servlet.ServletRequest;
import javax.servlet.ServletResponse;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.util.Map;
public class ThreadVariables {
private ServletContext app;
private HttpServletRequest request;
private HttpServletResponse response;
private TemplateInfo templateInfo;
private String viewFolder;
private GroovyClassLoader groovyClassLoader;
private Binding binding;
private Map<String, Route> routes;
private Sql sql;
private RequestError requestError;
private boolean isTemplateRequest;
private String templateFilePath;
private UserPrincipal userPrincipal;
private Jws<Claims> claims;
public ThreadVariables(ServletContext app) {
this.app = app;
this.requestError = new RequestError();
}
public ThreadVariables(ServletContext app, ServletRequest request, ServletResponse response, Map<String,Route> routes, Sql sql, TemplateInfo templateInfo, GroovyClassLoader groovyClassLoader) {
this.app = app;
this.request = (HttpServletRequest)request;
this.response = (HttpServletResponse)response;
this.templateInfo = templateInfo;
this.binding = binding;
this.groovyClassLoader = groovyClassLoader;
this.routes = routes;
this.sql = sql;
this.requestError = new RequestError();
try {
this.claims = JwtUtil.parseJwtClaims((HttpServletRequest) request);
}catch (Exception e){
}
}
public Jws<Claims> getClaims() {
return claims;
}
public HttpServletRequest getRequest() {
return request;
}
public HttpServletResponse getResponse() {
return response;
}
public String getViewFolder() {
return viewFolder;
}
public void setViewFolder(String viewFolder) {
this.viewFolder = viewFolder;
}
public Binding getBinding() {
return binding;
}
public TemplateInfo getTemplateInfo() {
if (templateInfo == null){
templateInfo = new TemplateInfo();
}
return templateInfo;
}
public GroovyClassLoader getGroovyClassLoader() {
return groovyClassLoader;
}
public ServletContext getApp() {
return app;
}
public Map<String, Route> getRoutes() {
return routes;
}
public Sql getSql() {
return sql;
}
public void setSql(Sql sql){
this.sql = sql;
}
public RequestError getRequestError() {
return requestError;
}
public boolean isTemplateRequest() {
return isTemplateRequest;
}
public void setTemplateRequest(boolean templateRequest) {
isTemplateRequest = templateRequest;
}
public String getTemplateFilePath() {
return templateFilePath;
}
public void setTemplateFilePath(String templateFilePath) {
this.templateFilePath = templateFilePath;
}
public UserPrincipal getUserPrincipal() {
return userPrincipal;
}
public void setUserPrincipal(UserPrincipal userPrincipal) {
this.userPrincipal = userPrincipal;
}
}
<file_sep>package sybrix.easygsp2.framework;
public class ThreadBag {
private static final ThreadLocal<ThreadVariables> _id = new ThreadLocal<ThreadVariables>() {
protected ThreadVariables initialValue() {
return null;
}
};
public static ThreadVariables get() {
return _id.get();
}
public static void set(ThreadVariables id) {
_id.set(id);
}
public static void remove() {
try {
if (_id.get().getSql() != null) {
_id.get().getSql().close();
}
} catch (Throwable e) {
//do nothing
}
try {
_id.set(null);
_id.remove();
} catch (Throwable e) {
//do nothing
}
}
}
<file_sep># easygsp-framework
Because I really hate Spring and really like Groovy
It's a great product but Roo and Boot both just added more annotations and confusion.
Spring supports groovy so you can write a controller that looks almost exactly like this Spring, but you can't escape Spring's annotations. It's so many...so so many.
####How is it different?
Quite honestly, from a dev's perspective, it's not that different. EasyGsp is designed for use with Groovy.
and has smaller ambitions than Spring. Spring inspired this framework but from this point on, let's stop talking about Spring.
As of now, EasyGsp supports 2 annotations. @Api and @Secured
I'm sure more will come but as of now that's it.
Here's a controller in EasyGsp:
```groovy
def ProfileController {
def get(id){
def db = SqlConnection(...)
// do some database stuff
new Profile(username:db.username, lastname:db.lastname)
}
def post(ProfileRequest profileRequest){
}
def post(ProfileRequest profileRequest){
"forward:/api/images?q=a"
}
def post(ProfileRequest profileRequest){
new ControllerResponse(forwarded:true, url:'/url/image')
}
}
```
<file_sep>plugins {
id 'groovy'
id 'maven-publish'
id 'java-library'
}
group = 'sybrix-easygsp2'
version = '1.0.0-SNAPSHOT'
repositories {
mavenCentral()
mavenLocal()
}
publishing {
publications {
myLibrary(MavenPublication) {
from components.java
}
}
}
java {
//withJavadocJar()
withSourcesJar()
}
dependencies {
compileOnly 'org.codehaus.groovy:groovy-all:3.0.5'
api 'com.sun.mail:javax.mail:1.5.5'
api 'com.squareup.okhttp3:okhttp:4.0.1'
api 'com.squareup.okhttp3:logging-interceptor:4.0.1'
compileOnly 'org.codehaus.groovy:groovy-all:3.0.5'
testImplementation 'org.codehaus.groovy:groovy-all:3.0.5'
api 'commons-fileupload:commons-fileupload:1.3.2'
api 'net.sourceforge.jregex:jregex:1.2_01'
api 'org.javassist:javassist:3.20.0-GA'
api 'org.reflections:reflections:0.9.10'
api 'commons-codec:commons-codec:1.9'
api 'com.fasterxml.jackson.core:jackson-core:2.8.0'
api 'com.fasterxml.jackson.core:jackson-annotations:2.8.0'
api 'com.fasterxml.jackson.core:jackson-databind:2.8.0'
api 'io.jsonwebtoken:jjwt:0.9.0'
api 'org.hibernate.validator:hibernate-validator:6.0.7.Final'
api 'org.mindrot:jbcrypt:0.4'
api 'org.slf4j:slf4j-ext:1.8.0-alpha2'
compileOnly 'javax.servlet:javax.servlet-api:3.1.0'
compileOnly group: 'org.apache.logging.log4j', name: 'log4j-api', version: '2.10.0'
compileOnly group: 'org.apache.logging.log4j', name: 'log4j-core', version: '2.10.0'
compileOnly group: 'javax.persistence', name: 'persistence-api', version: '1.0.2'
// api 'sybrix:easygsp-util:1.0.0'
}
apply plugin: 'idea'
<file_sep>package sybrix.easygsp2.util;
import java.math.BigDecimal;
public class NumberUtil {
public static Double toDbl(Object val, Double defaultVal) {
if (val == null) {
return defaultVal;
}
try {
return toDbl(val);
} catch (Exception e) {
return defaultVal;
}
}
public static Double toDbl(Object val) {
if (val == null || StringUtil.isEmpty(val.toString())) {
return null;
}
return Double.parseDouble(val.toString());
}
public static BigDecimal toBD(Object val) {
if (val == null || StringUtil.isEmpty(val.toString())) {
return null;
}
return new BigDecimal(val.toString());
}
public static BigDecimal toBD(Object val, BigDecimal defaultVal) {
if (val == null) {
return defaultVal;
}
try {
return toBD(val);
} catch (Exception e) {
return defaultVal;
}
}
public static Integer toInt(Object val, Integer defaultVal) {
if (val == null) {
return defaultVal;
}
try {
return toInt(val);
} catch (Exception e) {
return defaultVal;
}
}
public static Integer toInt(Object val) {
if (val == null || StringUtil.isEmpty(val.toString())) {
return null;
}
return Integer.parseInt(val.toString());
}
public static Long toLong(Object val, Long defaultValue) {
if (val == null) {
return defaultValue;
}
try {
return toLong(val);
} catch (Exception e) {
return defaultValue;
}
}
public static Long toLong(Object val) {
if (val == null || StringUtil.isEmpty(val.toString())) {
return null;
}
if (val instanceof Number) {
return ((Number) val).longValue();
} else {
return Long.parseLong(val.toString());
}
}
public static BigDecimal round(BigDecimal d, Integer scale) {
return d.setScale(scale, BigDecimal.ROUND_HALF_UP);
}
}
<file_sep>package sybrix.easygsp2.util;
import java.util.ArrayList;
import java.util.List;
/**
* Created by dsmith on 9/4/16.
*/
public class CollectionsUtil {
public static <T> List<T> toList(T[] methods) {
List<T> l = new ArrayList<T>();
if (methods != null) {
for (T m : methods) {
l.add(m);
}
}
return l;
}
public static <T> T[] toArray(List<T> list) {
T[] ary = (T[]) new Object[list.size()];
list.toArray(ary);
return ary;
}
public static <T> T[] add(T[] ary1, T[] ary2) {
int i = ary1 != null ? ary1.length : 0;
i = i + (ary2 != null ? ary2.length : 0);
T[] ary = (T[])new Object[i];
if (ary1!=null){
for(int x=0;x<ary1.length;x++){
ary[x] = ary1[x];
}
}
if (ary2!=null){
for(int x=0;x<ary2.length;x++){
ary[x] = ary2[x];
}
}
return ary;
}
}
<file_sep>package sybrix.easygsp2.exceptions;
public class NoViewTemplateFound extends RuntimeException {
public NoViewTemplateFound(String msg) {
super(msg);
}
}
| a39596fd3caf5573622f75c413d7613ca116f16e | [
"Markdown",
"Java",
"Gradle"
] | 14 | Java | sybrix/easygsp2-framework | 717939f2987e8e6bb6fec6cf46b57732eb47f37a | 7d8e6f8d914e770b0377253082f437fb79453d54 | |
refs/heads/master | <repo_name>davidyi0529/Speedquiz_D<file_sep>/script.js
function initQuiz() {
let timeRemaining=0;
let questions = [
{
title: "Question 1: What tag is used to specify a section of text that provides an example of computer code?",
choices: ["caption", "code", "!DOCTYPE", "embed"],
answer: "code"
},
{
title: "Question 2: What tag can be used to insert a line break or blank line in an HTML document?",
choices: ["br", "body", "head", "title"],
answer: "br"
},
{
title: "Question 3: What tag is used to define a standard cell inside a table?",
choices: ["td", "h1", "button", "footer"],
answer: "td"
},
{
title: "Question 4: What element is a container for all the head elements, and may include the document title, scripts, styles, meta information, and more?",
choices: ["head", "br", "body", "title"],
answer: "head"
},
{
title: "Question 5: What is the CSS property that is used to specify the edges of a table?",
choices: ["Fill", "Boxes", "Margins", "Borders"],
answer: "Borders"
},
{
title: "Question 6: What is the CSS property that sets the size of the whitespace outside the borders of the content?",
choices: ["Line", "Spacer", "Margin", "Block-level"],
answer: "Margin"
},
{
title: "Question 7: What is the name of the property used to specify the effects displayed behind all elements on a page?",
choices: ["Bottom Layer", "Background", "Border", "Transparency"],
answer: "Background"
},
{
title: "Question 8: What is the element used – and hidden – in code that explains things and makes the content more readable?",
choices: ["Comparisons", "Comments", "Quotations", "Notes"],
answer: "Comments"
},
{
title: "Question 9: What is the object called that lets you work with both dates and time-related data?",
choices: ["Dates", "Time-warp", "Time field", "Clock"],
answer: "Dates"
},
{
title: "Question 10: What is a JavaScript element that represents either TRUE or FALSE values?",
choices: ["Boolean", "RegExp", "Condition", "Event"],
answer: "Boolean"
}
];
let startButtonEl = document.getElementById("start-button");
let timeRemainingEl = document.getElementById("time-remaining");
let finalScoreEl = document.getElementById("final-score");
let numQuestions = questions.length;
let landingContainerEl = document.getElementById("landing-container");
let quizContainerEl = document.getElementById("quiz-container");
let finalContainerEl = document.getElementById("final-container");
let submitButtonEl = document.getElementById("submit-initials");
let highscoreButtonEl = document.getElementById("highscore-button");
let highscoreContainerEl = document.getElementById("highscore-container");
let highScores = [];
if (JSON.parse(localStorage.getItem('scores')) !== null) {
highScores = JSON.parse(localStorage.getItem("scores"));
}
function startQuiz() {
landingContainerEl.setAttribute("class","container d-none");
let rowEl = null;
let colEl = null;
let headerEl = null;
let buttonEl = null;
quizContainerEl.setAttribute("class","container");
let currentQuestion = 1;
let score = 0;
timeRemaining=numQuestions * 15;
timeRemainingEl.setAttribute("value",timeRemaining);
let myInterval = setInterval(function() {
if (timeRemaining<1) {
clearInterval(myInterval);
quizContainerEl.setAttribute("class","container d-none");
finalContainerEl.setAttribute("class","container");
return;
}
timeRemaining = timeRemaining - 1;
timeRemainingEl.setAttribute("value",timeRemaining);
},1000);
let clickTimeout = false;
function generateQuestion(questionNum) {
quizContainerEl.innerHTML = "";
rowEl = document.createElement("div");
rowEl.setAttribute("class","row");
quizContainerEl.append(rowEl);
colEl = document.createElement("div");
colEl.setAttribute("class","col-0 col-sm-2");
rowEl.append(colEl);
colEl = document.createElement("div");
colEl.setAttribute("class","col-12 col-sm-8");
rowEl.append(colEl);
colEl = document.createElement("div");
colEl.setAttribute("class","col-0 col-sm-2");
rowEl.append(colEl);
colEl = rowEl.children[1];
rowEl = document.createElement("div");
rowEl.setAttribute("class","row mb-3");
colEl.append(rowEl);
colEl = document.createElement("div");
colEl.setAttribute("class","col-12");
rowEl.append(colEl);
headerEl = document.createElement("h2");
headerEl.innerHTML = questions[questionNum-1].title;
colEl.append(headerEl);
colEl = quizContainerEl.children[0].children[1];
for (let i=0; i<4; i++) {
let rowEl = document.createElement("div");
rowEl.setAttribute("class","row mb-1");
colEl.append(rowEl);
let colEl2 = document.createElement("div");
colEl2.setAttribute("class","col-12");
rowEl.append(colEl2);
buttonEl = document.createElement("button");
buttonEl.setAttribute("class","btn btn-primary");
buttonEl.setAttribute("type","button");
buttonEl.innerHTML = questions[currentQuestion-1].choices[i];
colEl2.append(buttonEl);
buttonEl.addEventListener("click",function(){
if (clickTimeout) {
return;
}
clickTimeout = true;
clearInterval(myInterval);
let colEl = quizContainerEl.children[0].children[1];
let rowEl = document.createElement("div");
rowEl.setAttribute("class","row border-top");
colEl.append(rowEl);
colEl = document.createElement("div");
colEl.setAttribute("class","col-12");
rowEl.append(colEl);
let parEl = document.createElement("p");
colEl.append(parEl);
if (this.innerHTML === questions[currentQuestion - 1].answer) {
parEl.innerHTML = "Correct!";
} else {
parEl.innerHTML = "Incorrect";
timeRemaining = timeRemaining - 10;
if (timeRemaining < 0) {
timeRemaining = 0;
}
timeRemainingEl.setAttribute("value",timeRemaining);
}
currentQuestion++;
if (currentQuestion>questions.length) {
score = timeRemaining;
}
setTimeout(function() {
if (currentQuestion>questions.length) {
quizContainerEl.setAttribute("class","container d-none");
finalContainerEl.setAttribute("class","container");
finalScoreEl.setAttribute("value",score);
} else {
generateQuestion(currentQuestion);
clickTimeout = false;
myInterval = setInterval(function() {
if (timeRemaining<1) {
clearInterval(myInterval);
quizContainerEl.setAttribute("class","container d-none");
finalContainerEl.setAttribute("class","container");
return;
}
timeRemaining = timeRemaining - 1;
timeRemainingEl.setAttribute("value",timeRemaining);
},1000);
}
},1000);
});
}
}
function saveHighScore() {
let initialsEl = document.getElementById("initials-entry");
let newHighScore = {
initials: initialsEl.value,
highScore: score
};
console.log(newHighScore);
highScores.push(newHighScore);
console.log(highScores);
localStorage.setItem("scores",JSON.stringify(highScores));
}
submitButtonEl.addEventListener("click",saveHighScore);
generateQuestion(currentQuestion);
}
startButtonEl.addEventListener("click",startQuiz);
highscoreButtonEl.addEventListener("click",function() {
landingContainerEl.setAttribute("class","container d-none");
quizContainerEl.setAttribute("class","container d-none");
finalContainerEl.setAttribute("class","container d-none");
highscoreContainerEl.setAttribute("class","container");
let colEl = document.getElementById("highscore-table");
for (i=0; i<highScores.length; i++) {
let rowEl = document.createElement("div");
rowEl.setAttribute("class","row mb-1");
colEl.append(rowEl);
let colEl2 = document.createElement("div");
colEl2.setAttribute("class","col-12 text-center");
rowEl.append(colEl2);
let parEl = document.createElement("div");
parEl.innerHTML = "Initials: " + highScores[i].initials + " Score: " + highScores[i].highScore;
colEl2.append(parEl);
}
});
}
initQuiz();<file_sep>/README.md
<img src="https://github.com/davidyi0529/Portfolio_D/blob/master/assets/images/logo.png?raw=true" alt="alt text" title="<NAME>">
<br />
# Speedquiz_D
<br />
- [Speedquiz_D direct page Link](https://davidyi0529.github.io/Speedquiz_D/)
<br />
Speedquiz_D is a quiz that asks the user a series of questions about HTML, CSS, & Javascript and times how long it takes to answer them all. The user is given 150 seconds to start with, and each wrong answer results in a 10-second penalty. The user's final score is equal to the number of seconds left on the clock after the final question is answered.
To start the quiz, the user clicks the "Start Quiz" button on the landing page.
### Screenshot of landing page
<img src="https://github.com/davidyi0529/Speedquiz_D/blob/master/assets/images/landing%20page.png?raw=true" width="900" height="400">
<br />
<br />
Once the quiz is started, the user is presented with a question, along with 4 possible answers. Upon clicking one of the possible answers, the app will tell the user whether their answer was correct or not, deduct 10 seconds from the remaining time if incorrect, then load the next question.
### Screenshot of quiz page
<img src="https://github.com/davidyi0529/Speedquiz_D/blob/master/assets/images/quiz%20page.png?raw=true" width="900" height="400">
<br />
<br />
Once the final question is answered, the user's final score is shown, and the user can save their score upon entering their initials. When the "Submit" button is clicked, the initials and score are saved to the local storage, so even after the browser is refreshed the score will be saved. The list of saved scores can be seen by clicking the "View Hichscores" button.
### Screenshot of final page
<img src="https://github.com/davidyi0529/Speedquiz_D/blob/master/assets/images/final%20page.png?raw=true" width="900" height="400">
<br />
<br />
### Screenshot of highscore page
<img src="https://github.com/davidyi0529/Speedquiz_D/blob/master/assets/images/high%20score.png?raw=true" width="900" height="400">
<br />
<br />
---
<br />
<br />
## Table of Contents
- [Installation](#installation)
- [Features](#features)
- [Credits](#credits)
- [Support](#support)
- [License](#license)
<br />
<br />
---
<br />
<br />
## Installation
<br />
> Below I have shared a link to clone the repo, if perhaps you would like to see or contribute to the code.
<br />
### Clone
<br />
```bash
Clone this repo to your local machine using 'https://github.com/davidyi0529/Speedquiz_D.git'
```
<br />
<br />
---
<br />
<br />
## Features
<br />
<br />
The following were used for this project.
- `Javascript`
- `HTML`
- `Bootstrap`
<br />
Mainly Javascript was used for this project. This provided good practice in navigating the DOM in javascript, along with the use of `this` when creating a button and defining its on-click behavior to check its own text and compare to the correct answer.
A particularly fustrating problem was storing an array of objects into the local storage and then retrieving them. I was puzzled to get an error when I tried to add elements to this array saying *"myArray.push is not a function."* After doing some research I found that for arrays to behave properly you need to convert them to a string when you save them to the local storage, then convert them from a string back to an array when you retrieve them again.
<br />
<br />
---
<br />
<br />
## Credits
<br />
><a href="https://bootcamp.berkeley.edu/coding/" target="_blank">`UCB-Coding-Bootcamp`</a>
><a href="w3schools.com" target="_blank">`W3Schools`</a>
><a href="https://shields.io/ " target="_blank">`Shields.io`</a>
<br />
<br />
---
<br />
<br />
## Support
<br />
Reach out to me at one of the following places!
> Linkedin @ <a href="www.linkedin.com/in/davidyi0529" target="_blank">`davidyi0529`</a>
> Github @ <a href="https://github.com/davidyi0529" target="_blank">`davidyi0529`</a>
<br />
<br />
---
<br />
<br />
## License

- **[MIT license](http://opensource.org/licenses/mit-license.php)** | 2142661762b2f2596501ee574b3f7dc6be11f673 | [
"JavaScript",
"Markdown"
] | 2 | JavaScript | davidyi0529/Speedquiz_D | 0d357131fbf17537d5f1f83fd3039afe5c8867d7 | d7fbb477e3d014ab18621883a29fd323baabee8d | |
refs/heads/master | <repo_name>thatlisajones/Survey_Example<file_sep>/app/models/feelings.js
var feelings = [
{
"feeling_id": 1,
"feeling_group": "Joy",
"feeling_name":"Excited",
"feeling_emoji":"😁",
"feeling_color":"#ADFF2F",
"feeling_def": "Enthusiastic and eager",
"feeling_value":101
},
{
"feeling_id": 2,
"feeling_group": "Joy",
"feeling_name":"Sensuous",
"feeling_emoji":"😏",
"feeling_color":"#006400",
"feeling_def": "Physically attractive or attracted",
"feeling_value":102
},
{
"feeling_id": 3,
"feeling_group": "Joy",
"feeling_name":"Energetic",
"feeling_emoji":"🤪",
"feeling_color":"#228B22",
"feeling_def": "Showing great activity or vitality",
"feeling_value":103
},
{
"feeling_id": 4,
"feeling_group": "Joy",
"feeling_name":"Cheerful",
"feeling_emoji":"🌞",
"feeling_color":"#00FF00",
"feeling_def": "Noticeably happy or optimistic",
"feeling_value":104
},
{
"feeling_id": 5,
"feeling_group": "Joy",
"feeling_name":"Creative",
"feeling_emoji":"🖌",
"feeling_color":"#32CD32",
"feeling_def": "Full of desire to express imagination or ideas",
"feeling_value":105
},
{
"feeling_id": 6,
"feeling_group": "Joy",
"feeling_name":"Hopeful",
"feeling_emoji":"🤞",
"feeling_color":"#7CFC00",
"feeling_def": "Feeling or inspiring optimism about the future",
"feeling_value":106
},
{
"feeling_id": 7,
"feeling_group": "Joy",
"feeling_name":"Adventurous",
"feeling_emoji":"🍃",
"feeling_color":"#6B8E23",
"feeling_def": "Willing to take risks and try new things",
"feeling_value":107
},
{
"feeling_id": 8,
"feeling_group": "Joy",
"feeling_name":"Fascinated",
"feeling_emoji":"🧐",
"feeling_color":"#5DBF40",
"feeling_def": "Strongly attracted or interested",
"feeling_value":108
},
{
"feeling_id": 9,
"feeling_group": "Joy",
"feeling_name":"Stimulated",
"feeling_emoji":"😜",
"feeling_color":"#17FF0F",
"feeling_def": "Strongly motivated or invigorated",
"feeling_value":109
},
{
"feeling_id": 10,
"feeling_group": "Joy",
"feeling_name":"Helpful",
"feeling_emoji":"🤝",
"feeling_color":"#98FB98",
"feeling_def": "Useful or wanting to be useful to others",
"feeling_value":110
},
{
"feeling_id": 11,
"feeling_group": "Joy",
"feeling_name":"Playful",
"feeling_emoji":"🌼",
"feeling_color":"#8AFFA5",
"feeling_def": "Lighthearted and full of amusement",
"feeling_value":111
},
{
"feeling_id": 12,
"feeling_group": "Joy",
"feeling_name":"Delighted",
"feeling_emoji":"🤗",
"feeling_color":"#00A300",
"feeling_def": "Feeling or showing great pleasure",
"feeling_value":112
},
{
"feeling_id": 13,
"feeling_group": "Peace",
"feeling_name":"Calm",
"feeling_emoji":"😌",
"feeling_color":"#4B0082",
"feeling_def": "Free of nervousness or unsettling feelings",
"feeling_value":201
},
{
"feeling_id": 14,
"feeling_group": "Peace",
"feeling_name":"Fulfilled",
"feeling_emoji":"🙌",
"feeling_color":"#8B008B",
"feeling_def": "Satisfied because of fully developing one's abilities or character",
"feeling_value":202
},
{
"feeling_id": 15,
"feeling_group": "Peace",
"feeling_name":"Meditative",
"feeling_emoji":"🌴",
"feeling_color":"#9932CC",
"feeling_def": "Absorbed in contemplation or careful thought",
"feeling_value":203
},
{
"feeling_id": 16,
"feeling_group": "Peace",
"feeling_name":"Harmonious",
"feeling_emoji":"☮",
"feeling_color":"#9400D3",
"feeling_def": "Feeling part of a pleasing or consistent whole",
"feeling_value":204
},
{
"feeling_id": 17,
"feeling_group": "Peace",
"feeling_name":"Loving",
"feeling_emoji":"💝",
"feeling_color":"#8A2BE2",
"feeling_def": "Feeling or showing great care",
"feeling_value":205
},
{
"feeling_id": 18,
"feeling_group": "Peace",
"feeling_name":"Trusting",
"feeling_emoji":"🤲",
"feeling_color":"#9370DB",
"feeling_def": "Feeling a belief in honesty or sincerity; not suspicious",
"feeling_value":206
},
{
"feeling_id": 19,
"feeling_group": "Peace",
"feeling_name":"Nurturing",
"feeling_emoji":"🐱",
"feeling_color":"#BA55D3",
"feeling_def": "Cherishing or encouraging growth or development",
"feeling_value":207
},
{
"feeling_id": 20,
"feeling_group": "Peace",
"feeling_name":"Kind",
"feeling_emoji":"👭",
"feeling_color":"#FF00FF",
"feeling_def": "Feeling friendly and considerate",
"feeling_value":208
},
{
"feeling_id": 21,
"feeling_group": "Peace",
"feeling_name":"Validated",
"feeling_emoji":"✅",
"feeling_color":"#DA70D6",
"feeling_def": "Feeling supported or affirmed",
"feeling_value":209
},
{
"feeling_id": 22,
"feeling_group": "Peace",
"feeling_name":"Generous",
"feeling_emoji":"💜",
"feeling_color":"#EE82EE",
"feeling_def": "Ready to give more than necessary or expected",
"feeling_value":210
},
{
"feeling_id": 23,
"feeling_group": "Peace",
"feeling_name":"Secure",
"feeling_emoji":"🔐",
"feeling_color":"#DDA0DD",
"feeling_def": "Feeling safe, stable, and free from fear or worry",
"feeling_value":211
},
{
"feeling_id": 24,
"feeling_group": "Peace",
"feeling_name":"Thankful",
"feeling_emoji":"🙏",
"feeling_color":"#E6E6FA",
"feeling_def": "Feeling gratitude and relief",
"feeling_value":212
},
{
"feeling_id": 25,
"feeling_group": "Confidence",
"feeling_name":"Loyal",
"feeling_emoji":"💖",
"feeling_color":"#F0F8FF",
"feeling_def": "Giving or showing firm and constant support or allegiance",
"feeling_value":301
},
{
"feeling_id": 26,
"feeling_group": "Confidence",
"feeling_name":"Needed",
"feeling_emoji":"🐶",
"feeling_color":"#B0E0E6",
"feeling_def": "Feeling essential or necessary",
"feeling_value":302
},
{
"feeling_id": 27,
"feeling_group": "Confidence",
"feeling_name":"Appreciated",
"feeling_emoji":"💎",
"feeling_color":"#87CEFA",
"feeling_def": "Feeling that one's full worth is recognized",
"feeling_value":303
},
{
"feeling_id": 28,
"feeling_group": "Confidence",
"feeling_name":"Respected",
"feeling_emoji":"🏆",
"feeling_color":"#87CEEB",
"feeling_def": "Feeling admired for one's abilities, qualities, or achievements",
"feeling_value":304
},
{
"feeling_id": 29,
"feeling_group": "Confidence",
"feeling_name":"Dignified",
"feeling_emoji":"👑",
"feeling_color":"#00BFFF",
"feeling_def": "Having or showing a composed or serious manner that is worthy of respect",
"feeling_value":305
},
{
"feeling_id": 30,
"feeling_group": "Confidence",
"feeling_name":"Blessed",
"feeling_emoji":"🎁",
"feeling_color":"#1E90FF",
"feeling_def": "Feeling endowed with divine favor and protection",
"feeling_value":306
},
{
"feeling_id": 31,
"feeling_group": "Confidence",
"feeling_name":"Lucky",
"feeling_emoji":"🍀",
"feeling_color":"#6495ED",
"feeling_def": "Feeling favored by chance or fortune",
"feeling_value":307
},
{
"feeling_id": 32,
"feeling_group": "Confidence",
"feeling_name":"Discerning",
"feeling_emoji":"🧐",
"feeling_color":"#4682B4",
"feeling_def": "Having or showing good judgement",
"feeling_value":308
},
{
"feeling_id": 33,
"feeling_group": "Confidence",
"feeling_name":"Loved",
"feeling_emoji":"😍",
"feeling_color":"#7B68EE",
"feeling_def": "Feeling deeply cared for",
"feeling_value":309
},
{
"feeling_id": 34,
"feeling_group": "Confidence",
"feeling_name":"Admirable",
"feeling_emoji":"😇",
"feeling_color":"#6A5ACD",
"feeling_def": "Deserving of respect or approval",
"feeling_value":310
},
{
"feeling_id": 35,
"feeling_group": "Confidence",
"feeling_name":"Successful",
"feeling_emoji":"🥇",
"feeling_color":"#4169E1",
"feeling_def": "Feeling of accomplishment or achievement",
"feeling_value":311
},
{
"feeling_id": 36,
"feeling_group": "Confidence",
"feeling_name":"Deserving",
"feeling_emoji":"💯",
"feeling_color":"#0000CD",
"feeling_def": "Feeling worthy of good treatment or assistance",
"feeling_value":312
},
{
"feeling_id": 37,
"feeling_group": "Sadness",
"feeling_name":"Sorry",
"feeling_emoji":"😰",
"feeling_color":"#FF4500",
"feeling_def": "Feeling regret or distress, especially through sympathy with someone else.",
"feeling_value":401
},
{
"feeling_id": 38,
"feeling_group": "Sadness",
"feeling_name":"Stupid",
"feeling_emoji":"🤯",
"feeling_color":"#F7A036",
"feeling_def": "Feeling annoyed or inadequate due to one's mistakes or lack of sense",
"feeling_value":402
},
{
"feeling_id": 39,
"feeling_group": "Sadness",
"feeling_name":"Inferior",
"feeling_emoji":"🥉",
"feeling_color":"#F2AC92",
"feeling_def": "Feeling disadvantaged, less-than, or of lower status",
"feeling_value":403
},
{
"feeling_id": 40,
"feeling_group": "Sadness",
"feeling_name":"Isolated",
"feeling_emoji":"😔",
"feeling_color":"#DAB68B",
"feeling_def": "Having minimal contact or little in common with others",
"feeling_value":404
},
{
"feeling_id": 41,
"feeling_group": "Sadness",
"feeling_name":"Uninterested",
"feeling_emoji":"💤",
"feeling_color":"#E6830A",
"feeling_def": "Not caring about something or someone",
"feeling_value":405
},
{
"feeling_id": 42,
"feeling_group": "Sadness",
"feeling_name":"Disappointed",
"feeling_emoji":"😞",
"feeling_color":"#FFD8A8",
"feeling_def": "Unhappy because someone or something did not fulfill one's hopes or expectations",
"feeling_value":406
},
{
"feeling_id": 43,
"feeling_group": "Sadness",
"feeling_name":"Guilty",
"feeling_emoji":"☣",
"feeling_color":"#FF8C00",
"feeling_def": "Feeling responsible for wrongdoing",
"feeling_value":407
},
{
"feeling_id": 44,
"feeling_group": "Sadness",
"feeling_name":"Ashamed",
"feeling_emoji":"😓",
"feeling_color":"#FF6347",
"feeling_def": " Feeling embarrassed or guilty because of one's actions, characteristics, or associations",
"feeling_value":408
},
{
"feeling_id": 45,
"feeling_group": "Sadness",
"feeling_name":"Depressed",
"feeling_emoji":"😭",
"feeling_color":"#FFD4CC",
"feeling_def": "Feeling general unhappiness or despondency",
"feeling_value":409
},
{
"feeling_id": 46,
"feeling_group": "Sadness",
"feeling_name":"Lonely",
"feeling_emoji":"🥀",
"feeling_color":"#F4AC9F",
"feeling_def": "Feeling sad because one has no friends or company",
"feeling_value":410
},
{
"feeling_id": 47,
"feeling_group": "Sadness",
"feeling_name":"Bored",
"feeling_emoji":"🐗",
"feeling_color":"#FF7F50",
"feeling_def": "Feeling weary because one is unoccupied or lacks interest in one's current activity",
"feeling_value":411
},
{
"feeling_id": 48,
"feeling_group": "Sadness",
"feeling_name":"Tired",
"feeling_emoji":"😴",
"feeling_color":"#FFCEBD",
"feeling_def": "In need of sleep or rest",
"feeling_value":412
},
{
"feeling_id": 49,
"feeling_group": "Anger",
"feeling_name":"Critical",
"feeling_emoji":"🔍",
"feeling_color":"#FF6347",
"feeling_def": "Expressing adverse or disapproving comments or judgments",
"feeling_value":501
},
{
"feeling_id": 50,
"feeling_group": "Anger",
"feeling_name":"Spiteful",
"feeling_emoji":"😒",
"feeling_color":"#FF4500",
"feeling_def": "Feeling malice or nastiness toward someone or something",
"feeling_value":502
},
{
"feeling_id": 51,
"feeling_group": "Anger",
"feeling_name":"Outraged",
"feeling_emoji":"🤬",
"feeling_color":"#8B0000",
"feeling_def": "Fierce anger, shock, or indignation",
"feeling_value":503
},
{
"feeling_id": 52,
"feeling_group": "Anger",
"feeling_name":"Bitter",
"feeling_emoji":"☹",
"feeling_color":"#B22222",
"feeling_def": "Angry, hurt, or resentful because of one's bad experiences or a sense of unjust treatment",
"feeling_value":504
},
{
"feeling_id": 53,
"feeling_group": "Anger",
"feeling_name":"Resentful",
"feeling_emoji":"😤",
"feeling_color":"#DC143C",
"feeling_def": "Feeling aggrieved at having been treated unfairly",
"feeling_value":505
},
{
"feeling_id": 54,
"feeling_group": "Anger",
"feeling_name":"Hurt",
"feeling_emoji":"😢",
"feeling_color":"#CD5C5C",
"feeling_def": "Feeling mental pain or distress",
"feeling_value":506
},
{
"feeling_id": 55,
"feeling_group": "Anger",
"feeling_name":"Skeptical",
"feeling_emoji":"🤨",
"feeling_color":"#F08080",
"feeling_def": "Not easily convinced; having doubts or reservations",
"feeling_value":507
},
{
"feeling_id": 56,
"feeling_group": "Anger",
"feeling_name":"Irritated",
"feeling_emoji":"😖",
"feeling_color":"#E9967A",
"feeling_def": "Feeling slight anger or annoyance",
"feeling_value":508
},
{
"feeling_id": 57,
"feeling_group": "Anger",
"feeling_name":"Frustrated",
"feeling_emoji":"😣",
"feeling_color":"#990000",
"feeling_def": "Feeling or expressing distress and annoyance, especially because of inability to change or achieve something",
"feeling_value":509
},
{
"feeling_id": 58,
"feeling_group": "Anger",
"feeling_name":"Dismissive",
"feeling_emoji":"🙄",
"feeling_color":"#FFADAD",
"feeling_def": "Feeling or showing that something is unworthy of consideration",
"feeling_value":510
},
{
"feeling_id": 59,
"feeling_group": "Anger",
"feeling_name":"Distant",
"feeling_emoji":"🏔",
"feeling_color":"#FA8072",
"feeling_def": "Not intimate; cool or reserved",
"feeling_value":511
},
{
"feeling_id": 60,
"feeling_group": "Anger",
"feeling_name":"Jealous",
"feeling_emoji":"😠",
"feeling_color":"#8B0000",
"feeling_def": "Feeling envy of someone or their achievements and advantages; feeling suspicion of someone's unfaithfulness in a relationship",
"feeling_value":512
},
{
"feeling_id": 61,
"feeling_group": "Fear",
"feeling_name":"Confused",
"feeling_emoji":"😕",
"feeling_color":"#FFFFCC",
"feeling_def": "Feeling bewildered or unable to understand",
"feeling_value":601
},
{
"feeling_id": 62,
"feeling_group": "Fear",
"feeling_name":"Rejected",
"feeling_emoji":"❌",
"feeling_color":"#FFFF99",
"feeling_def": "Feeling dismissed as inadequate, inappropriate, or not preferred.",
"feeling_value":602
},
{
"feeling_id": 63,
"feeling_group": "Fear",
"feeling_name":"Helpless",
"feeling_emoji":"🤷",
"feeling_color":"#FFFF66",
"feeling_def": "Unable to defend oneself or to act without help",
"feeling_value":603
},
{
"feeling_id": 64,
"feeling_group": "Fear",
"feeling_name":"Belittled",
"feeling_emoji":"😲",
"feeling_color":"#FFFF33",
"feeling_def": "Feeling trivialized or downgraded by someone else",
"feeling_value":604
},
{
"feeling_id": 65,
"feeling_group": "Fear",
"feeling_name":"Insecure",
"feeling_emoji":"😳",
"feeling_color":"#FFFF00",
"feeling_def": "Not confident or assured; uncertain and anxious",
"feeling_value":605
},
{
"feeling_id": 66,
"feeling_group": "Fear",
"feeling_name":"Anxious",
"feeling_emoji":"😰",
"feeling_color":"#FFFFE0",
"feeling_def": "Worry, unease, or nervousness, typically about an imminent event or something with an uncertain outcome",
"feeling_value":606
},
{
"feeling_id": 67,
"feeling_group": "Fear",
"feeling_name":"Overwhelmed",
"feeling_emoji":"🌋",
"feeling_color":"#FFFACD",
"feeling_def": "Engulfed, defeated, or paralyzed by too much feeling",
"feeling_value":607
},
{
"feeling_id": 68,
"feeling_group": "Fear",
"feeling_name":"Embarrassed",
"feeling_emoji":"🤭",
"feeling_color":"#EEE8AA",
"feeling_def": "Feeling awkward, self-conscious, or ashamed",
"feeling_value":608
},
{
"feeling_id": 69,
"feeling_group": "Fear",
"feeling_name":"Inadequate",
"feeling_emoji":"⭕",
"feeling_color":"#FAFAD2",
"feeling_def": "Feeling unable to deal with a situation or with life",
"feeling_value":609
},
{
"feeling_id": 70,
"feeling_group": "Fear",
"feeling_name":"Insignificant",
"feeling_emoji":"🐀",
"feeling_color":"#CCCC00",
"feeling_def": "Feeling too small or unimportant to be worth consideration",
"feeling_value":610
},
{
"feeling_id": 71,
"feeling_group": "Fear",
"feeling_name":"Discouraged",
"feeling_emoji":"😟",
"feeling_color":"#F0F093",
"feeling_def": "Having lost confidence or enthusiasm; disheartened",
"feeling_value":611
},
{
"feeling_id": 72,
"feeling_group": "Fear",
"feeling_name":"Frightened",
"feeling_emoji":"😱",
"feeling_color":"#FFFF85",
"feeling_def": "Feeling deterred from involvement or action; strong fear or anxiety.",
"feeling_value":612
}
]
module.exports = feelings;
<file_sep>/app/routing/apiRoutes.js
// var feelingsData = require("../data/feelings");
// //GET route for url /api/feelings -- displays a JSON of all possible feelings
// module.exports = function(app) {
// app.get("/api/feelings", function(req, res) {
// return res.json(feelingsData);
// });
// //POST route /api/feelings -- handles incoming survey results and compatibility logic
// app.post("/api/feelings", function(req, res) {
// var newfeeling = req.body;
// console.log(newfeeling);
// //add code for the feeling matching based on new feeling details
// newfeeling.routeName = newfeeling.name.replace(/\s+/g, "").toLowerCase();
// console.log(`${newfeeling.name} has been added to Fakefeeling Finder.`);
// feelings.push(newfeeling);
// res.json(newfeeling);
// })};
var feelings = require("../data/feelings");
module.exports = function(app) {
// Return all feelings found in feelings.js as JSON
app.get("/api/feelings", function(req, res) {
res.json(feelings);
});
app.post("/api/feelings", function(req, res) {
console.log("req.body: ", req.body);
console.log("body of post questions: ",req.body.questions);
// Receive user details (name, photo, questions)
var user = req.body;
// parseInt for questions
for(var i = 0; i < user.questions.length; i++) {
user.questions[i] = parseInt(user.questions[i]);
}
// default feeling match is the first feeling but result will be whoever has the minimum difference in questions
var compatibilityValue = 0;
var minimumDifference = 40;
// in this for-loop, start off with a zero difference and compare the user and the ith feeling questions, one set at a time
// whatever the difference is, add to the total difference
for(var i = 0; i < feelings.length; i++) {
var totalDifference = 0;
for(var j = 0; j < feelings[i].questions.length; j++) {
var difference = Math.abs(user.questions[j] - feelings[i].questions[j]);
totalDifference += difference;
}
// if there is a new minimum, change the best feeling index and set the new minimum for next iteration comparisons
if(totalDifference < minimumDifference) {
compatibilityValue = i;
minimumDifference = totalDifference;
}
}
// after finding match, add user to feeling array
feelings.push(user);
// send back to browser the best feeling match
res.json(feelings[compatibilityValue]);
});
}; | 64f31794139e747a6a6014f3a0cab53a1ebfc32d | [
"JavaScript"
] | 2 | JavaScript | thatlisajones/Survey_Example | cf6bb9228badf0418483c48ef8f10745e7cc911b | d956d29e9dac8af700c1211fb3220770a9bb7f93 | |
refs/heads/master | <file_sep>import React, { Component } from "react";
import { Link } from "react-router-dom";
// let url = window.location.href.split('/');
// let path = window.location.pathname;
class Navbar extends Component {
constructor() {
super();
this.state = {};
}
render() {
let { pathname } = this.props.location;
return (
<div className="navigation">
<div className="inner-nav">
<Link to="/" className={"/" === pathname ? "active" : "inactive"}>
About Me
</Link>
<Link
to="/languages"
className={"/languages" === pathname ? "active" : "inactive"}
>
Languages & Frameworks
</Link>
<Link
to="/projects"
className={"/projects" === pathname ? "active" : "inactive"}
>
My Projects
</Link>
<Link
to="contact"
className={"/contact" === pathname ? "active" : "inactive"}
>
Contact Me
</Link>
</div>
{/* {console.log(pathname)} */}
</div>
);
}
}
export default Navbar;
<file_sep>import React from "react";
import wise from "../images/projects/wise-code.png";
import geek from "../images/projects/geektext.png";
import rhaz from "../images/projects/rhaz.png";
import { FontAwesomeIcon } from "@fortawesome/react-fontawesome";
import { faLink, faCode } from "@fortawesome/free-solid-svg-icons";
const Projects = props => (
<div className="projects">
<div className="project1">
<div className="flip">
<div className="front">
<img src={wise} alt="wise-code" />
</div>
<div className="back">
<div className="project-name">
<strong>Wise Code - Mobile</strong>
</div>
<div className="contributions">
My Contributions:
<ul>
<li>
Implemented and maintained Graphql API for data management app
(WiseDB).
</li>
<li>Successfully created scripts to find and fix faulty data.</li>
<li>
Helped improve mutation efficiency and code cleanliness
(refactoring).
</li>
</ul>
</div>
<div className="tech">
Technologies used:
<ul>
<li>Graphql</li>
<li>Prisma</li>
<li>Yoga</li>
<li>Redis</li>
<li>Kubernetes</li>
<li>Docker</li>
</ul>
</div>
<div className="code-link">
<button className="project-link">
<FontAwesomeIcon icon={faLink} />
</button>
<button className="project-link">
<FontAwesomeIcon icon={faCode} />
</button>
</div>
</div>
</div>
</div>
<div className="project2">
<div className="flip">
<div className="front">
<img src={rhaz} alt="rhaz" />
</div>
<div className="back">
<div className="project-name">
<strong>RHAZ</strong>
</div>
<div className="contributions">
My Contributions:
<ul>
<li>Implemented logic for alien movement and re-generation.</li>
</ul>
</div>
<div className="tech">
Technologies used:
<ul>
<li>Unity</li>
<li>C#</li>
</ul>
</div>
<div className="code-link">
<button className="project-link">
<FontAwesomeIcon icon={faLink} />
</button>
<button className="project-link">
<FontAwesomeIcon icon={faCode} />
</button>
</div>
</div>
</div>
</div>
<div className="project3">
<div className="flip">
<div className="front coming-soon"></div>
<div className="back">COMING SOON</div>
</div>
</div>
<div className="project4">
<div className="flip">
<div className="front">
<img src={geek} alt="geek-text" />
</div>
<div className="back">
<div className="contributions-long">
<strong>Geek Text</strong>
<br />
<br />
My Contributions:
<ul>
<li>Implemented login feature.</li>
<li>Impremented create-account feature.</li>
<li>Used Stripe API for payment storage/verification.</li>
</ul>
</div>
<div className="tech-long">
Technologies used:
<ul>
<li>ReactJS</li>
<li>Java Springboot</li>
<li>Postgresql</li>
</ul>
</div>
<div className="code-link-long">
<button className="project-link">
<FontAwesomeIcon icon={faLink} />
</button>
<button className="project-link">
<FontAwesomeIcon icon={faCode} />
</button>
</div>
</div>
</div>
</div>
</div>
);
export default Projects;
<file_sep>import React, { Component } from "react";
import Navbar from "./components/Navbar";
import AboutMe from "./components/AboutMe";
import { BrowserRouter, Route, withRouter } from "react-router-dom";
import Languages from "./components/Languages";
import Projects from "./components/Projects";
class App extends Component {
constructor() {
super();
this.state = {
currentPage: window.location.pathname
};
}
render() {
return (
<BrowserRouter>
<div className="box">
<Route
path="/"
render={withRouter(props => (
<Navbar {...props} />
))}
/>
<Route exact path="/" component={AboutMe} />
<Route path="/languages" component={Languages} />
<Route path="/projects" component={Projects} />
</div>
{console.log("URL", process.env.PUBLIC_URL)}
</BrowserRouter>
);
}
}
export default App;
<file_sep>import React from "react";
import computer from "../images/computer.jpeg";
import { FontAwesomeIcon } from "@fortawesome/react-fontawesome";
import { faFileDownload } from "@fortawesome/free-solid-svg-icons";
import { faLinkedin, faGithub } from "@fortawesome/free-brands-svg-icons";
const AboutMe = props => (
<div className="content">
<div className="computer">
<img src={computer} alt="computer" />
</div>
<h1 className="name">
Hello, <br />
my name is <NAME>.
</h1>
<p className="about">
I currently work as a <strong>full-stack software developer</strong> and
enjoy challenging tasks, team collaboration and added responsibility. I am
a problem solver working to constantly expand my knowledge. I am
self-driven, detail- oriented, adaptable, and coachable, and hope to get
the chance to work on high-impact projects.
</p>
<div className="icons">
<button className="resume">
<FontAwesomeIcon icon={faFileDownload} />
</button>
<button
className="linkedin"
onClick={() => {
window.location.href =
"https://www.linkedin.com/in/janeth-arriola-a01463158/";
}}
>
<FontAwesomeIcon icon={faLinkedin} />
</button>
<button
className="github"
onClick={() => {
window.location.href = "https://github.com/Jarriola989";
}}
>
<FontAwesomeIcon icon={faGithub} />
</button>
</div>
</div>
);
export default AboutMe;
<file_sep>import React from "react";
import cplusplus from "../images/c++.png";
import graphql from "../images/graphql.png";
import javascript from "../images/javascript.png";
import typescript from "../images/typescript.png";
import java from "../images/java.png";
import html from "../images/html.png";
import css from "../images/css.png";
import sql from "../images/sql.png";
const Languages = props => (
<div className="languages">
<div className="java">
<img src={java} alt="java" />
</div>
<div className="javascript">
<img src={javascript} alt="javascript" />
</div>
<div className="typescript">
<img src={typescript} alt="typescript" />
</div>
<div className="html">
<img src={html} alt="html" />
</div>
<div className="css">
<img src={css} alt="css" />
</div>
<div className="graphql">
<img src={graphql} alt="graphql" />
</div>
<div className="sql">
<img src={sql} alt="sql" />
</div>
<div className="cplusplus">
<img src={cplusplus} alt="C++" />
</div>
</div>
);
export default Languages;
| 1c44d8700a634c5cf2b9c90b5f8757cab38f5a4d | [
"JavaScript"
] | 5 | JavaScript | Jarriola989/jarriola989.github.io | 63eff5092286398e941bc21f079c7603c27d8b09 | b87afb14444f06efdd33507312f12915897c8a8d | |
refs/heads/master | <repo_name>milo-trujillo/CriticalityTest<file_sep>/README.md
# Criticality Test
*<NAME>*
The Internet is a hybrid system - at a high level it is widely decentralized and redundant, but at local levels the network topology is often very rigidly structured. But how centralized *is* our network connection? How many ‘hops’ out to the Internet are critical hubs, any one of which failing would cut us off from the wider world?
This is a tool for auto-detecting the degree of local network centralization. This tool will explore routing information to reach a number of geographically diverse servers (three across the United States, more in Japan, India, Australia, and England). In a decentralized network the routes to reach each of these systems would be completely different. However, by identifying the overlaps between these routes we can determine how many systems are “critical hubs” all of our traffic must flow through before reaching the larger web.
To run the test:
sudo ./test.py
Results will appear in a few minutes after the traceroutes complete. Creating raw packets requires superuser access, so this tool must be run with `sudo`.
**NOTE**
At present this tool is only known to work on OSX. It will probably run correctly on any BSD system. However, it does *not* work on Linux systems. I think the Linux kernel steps on one of the IP or UDP headers I create and overrides information I was using for tracking responses.
<file_sep>/test.py
#!/usr/bin/env python
import socket, threading, random
from traceroute import traceroute
"""
The following hosts were chosen because we confirmed that their webhosting
is located at the locations their names specify. That is, we send a
traceroute to:
- Seattle, Washington
- Austin, Texas
- Portland, Maine
- London, UK
- Melbourne, Australia
- Tokyo, Japan
- Shakarpur, India
These destinations are geographically diverse enough that the routes taken
should significantly differ to reach at least one.
"""
Hosts = ["www.seattle.gov", "austintexas.gov", "www1.maine.gov", "cityoflondon.gov.uk", "australia.gov.au", "www.jnto.go.jp", "india.gov.in"]
Timeout = 120 # Maximum seconds to spend reaching any destination
BasePort = 33434 # Traceroute uses ports 33434 through 33534
def column(matrix, i):
return [row[i] for row in matrix]
# This finds the common hosts at the start of every route
def findCommonHops(routes):
if( len(routes) == 0 ):
return []
# Find the shortest route - we can't have any critical hops past that length
minLen = len(routes[0])
for r in routes:
if( len(r) < minLen ):
minLen = len(r)
commonHops = []
for i in range(0, minLen):
col = column(routes, i)
match = all(x == col[0] for x in col)
if( match ):
commonHops += [col[0]]
else:
return commonHops
return commonHops
# Reverse lookup, get a domain name for an IP address if possible
def getHostname(IP):
try:
name, alias, addresslist = socket.gethostbyaddr(host)
return name
except (socket.error, socket.herror, socket.gaierror, socket.timeout):
return "Unknown Host"
if __name__ == "__main__":
routes = []
routeLock = threading.RLock()
threads = []
random.seed()
print "Initating test. Spinning up traceroutes to %d destinations." % (len(Hosts))
#for i in range(0, 1):
for i in range(0, len(Hosts)):
host = Hosts[i]
# Choose a random high port that's unlikely to be listened to
port = random.randint(33434, 65500)
worker = threading.Thread(
target=traceroute,
args=(host, port, routes, routeLock))
threads += [worker]
worker.start()
print "Please be patient, it can take a few minutes to collect our data..."
for i in range(0, len(threads)):
threads[i].join()
print ""
print "================================================"
print "All traceroutes complete. Summary is as follows."
print "================================================"
print ""
common = findCommonHops(routes)
print "Leading common hosts in all routes: %d" % (len(common))
for host in common:
name = getHostname(host)
print "%s\t(%s)" % (host, name)
<file_sep>/traceroute.py
#!/usr/bin/env python
import socket, select, copy, struct
# Maximum TTL before we abort
MaxHops = 30
# How many seconds before we abort a certain TTL step
Timeout = 5
# If debug is enabled we print every step as received
Debug = False
"""
Traceroute implementation based on:
https://blogs.oracle.com/ksplice/entry/learning_by_doing_writing_your
However, unlike that example I implement the black magic used in BSD
to solve the multiple copies of traceroute problem.
You can read what I mean in the FreeBSD source code:
/usr/src/contrib/traceroute/traceroute.c
I use random numbers instead of PIDs, but it's the same principle.
I also used example code to parse the ICMP header myself:
https://gist.github.com/pklaus/856268
"""
def traceroute(host, port, routes, lock):
dest = socket.gethostbyname(host)
icmp = socket.getprotobyname('icmp')
udp = socket.getprotobyname('udp')
ttl = 1
route = []
with lock:
print "Attempting to reach %s at %s using port %d" % (host, dest, port)
while True:
recv_socket = None
send_socket = None
try:
recv_socket = socket.socket(socket.AF_INET, socket.SOCK_RAW, icmp)
#send_socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM, udp)
# Luke, you switched off your targeting computer! What's wrong?
# Nothing. I'm alright. Just writing all my packets by hand.
send_socket = socket.socket(socket.AF_INET, socket.SOCK_RAW, socket.IPPROTO_RAW)
except socket.error:
print "Unable to create raw socket. Try running as root?"
return
# Set up our sockets and send a UDP probe to our next hop
try:
send_socket.setsockopt(socket.SOL_IP, socket.IP_TTL, ttl)
recv_socket.bind(("", port + ttl))
send_socket.bind(("", port + ttl)) # THIS IS ESSENTIAL FOR BLACK MAGIC
probe = makeProbe(port + ttl, ttl, dest, port + ttl, port + ttl)
send_socket.sendto(probe, (dest, port + ttl))
except socket.error:
print "Unable to bind to port %d, perhaps it's already in use?" % (port)
return
# Okay, now let's try to receive an ICMP echo response for that UDP probe
# This may or may not succeed, depending on the host we sent to
addr = None
try:
# We use select to enforce a timeout. We also need to ignore packets
# destined for other traceroute instances.
while( True ):
ready = select.select([recv_socket], [], [], Timeout)
if( ready[0] ):
# Blocksize doesn't matter much, we use 512
data, src = recv_socket.recvfrom(512)
n = len(data)
sig = getICMPid(data)
src = src[0] # Extract just IP, not port
if( Debug ):
print "Read %d bytes from %s with signature: %s" % (n, src, sig)
if( sig >= port and sig <= port + MaxHops ):
addr = src
break
else:
break
except socket.error:
pass
finally:
send_socket.close()
recv_socket.close()
# Okay, at this point we're done trying to receive a packet.
# We either got an appropriate one from 'addr', or we hit
# our timeout and 'addr' is set to None.
if( addr is None ):
addr = "*"
if( Debug ):
print "%d\t%s" % (ttl, addr)
# Record the newly found hop in the route.
route += [copy.deepcopy(addr)]
ttl += 1
# If we're done then record our route in the global routing list
if( addr == dest or ttl > MaxHops ):
with lock:
routes += [route]
print "Host '%s' reached. Hops: %d" % (host, ttl)
if( Debug ):
print routeToString(route)
break
def routeToString(route):
s = ""
for r in route:
s += (str(r) + ",")
if( len(s) > 0 ):
return s[:-1]
return ""
"""
I read how to make a raw IP header from:
https://stackoverflow.com/questions/24520799
"""
def makeProbe(_id, ttl, dest, srcPrt, dstPrt):
version = 4 # IPv4
ihl = 5 # Header length in 32-bit words (5 words == 20 bytes)
DF = 0 # Optional fields, leaving them blank
# Length will be filled in by kernel
Tlen = 0 # Length of data (0) + 20 bytes for ipv4 header + 8 byte udp
ID = _id # ID of packet
Flag = 0 # Flags and fragment offset
TTL = ttl # Time to live
Proto = socket.IPPROTO_UDP # Protocol 17, UDP
ip_checksum = 0 # Checksum will be automatically filled in
SIP = socket.inet_aton("0.0.0.0")
DIP = socket.inet_aton(dest)
ver_ihl = ver_ihl = (version << 4) + ihl
#f_f = (Flag << 13) + Fragment
#ip_hdr = struct.pack("!BBHHHBBH4s4s", ver_ihl,DF,Tlen,ID,f_f,TTL,Proto,ip_checksum,SIP,DIP)
#return ip_hdr
IP_HEADER = struct.pack(
"!" # Network (Big endian)
"2B" # Version and IHL, DSCP, and ECN
"3H" # Total length, ID, Flags and fragment offset
"2B" # TTL, protocol
"H" # Checksum
"4s" # Source IP
"4s" # Dest IP
, ver_ihl, DF, Tlen, ID, Flag, TTL, Proto, ip_checksum, SIP, DIP)
# UDP uses "source port, dest port, length, checksum
UDP_HEADER = struct.pack("!4H", srcPrt, dstPrt, 8, 0)
return IP_HEADER + UDP_HEADER
"""
This parses the header of an ICMP packet and returns the ID
Heavily draws from https://gist.github.com/pklaus/856268
"""
def getICMPid(packet):
icmp_header = packet[20:]
_type, code = struct.unpack('bb', icmp_header[:2])
#_type, code, checksum, unused = struct.unpack('bbHL', icmp_header[:8])
# We only care about responses to traceroute (type 11 code 0)
if( _type != 11 ):
return -1
# ICMP header for type 11 will be:
# Type / code (2 bytes)
# Checksum (2 bytes)
# Unused (4 bytes)
#
# That's a total of 8 bytes used. After that we have:
# IP header (20 bytes?)
# 8 Bytes of UDP header data
# So if we just care about the UDP part we should skip 48 bytes in, for:
# Original IP header (20) + ICMP header (8) + 2nd IP header (20)
# The above math is a little off. By manual examination of the packets
# in wireshark we know we need to scroll in 52, not 48. Why? Magic.
return struct.unpack_from('!H', packet, 52)[0]
| e4e2999df6c02a5ff48d954bff97fb7f0054dc6e | [
"Markdown",
"Python"
] | 3 | Markdown | milo-trujillo/CriticalityTest | 8e8aeb4dd25b155363042dd2b63b8a57b58f86e5 | edda9c2f53ffb0f2d27d3853c536faf557dab2d7 | |
refs/heads/master | <file_sep># Runway-React
The `runway-react` package defines a `Gallery` component, which renders a thumbnail gallery and [Lightbox](https://github.com/rcwbr/react-images) viewer to display images processed by the `runway-gallery` tool.
## Usage
Example usage of the `runway-react Gallery` component can be found in the [test-runway](../test-runway/README.md) package.
The simplest use case of the `runway-react Gallery` component passes only the config prop:
```JavaScript
import Runway from 'runway-react'
<Runway config={runwayConfig} />
```
In this case, the `Gallery` defaults to render only the thumbnail gallery, with no Lightbox. `runwayConfig` is an object representation of the output of the `runway-gallery` tool, usually passed through the build tool. Here is an example using the [`runway-webpack-loader`](../runway-webpack-loader/README.md):
```JavaScript
import Runway from 'runway-react'
import runwayConfig from 'runway-webpack-loader!./gallery.runwayconf.json'
<Runway config={runwayConfig} />
```
Here, `gallery.runwayconf.json` would be a config file used as input to the `runway-gallery` tool. It could have this content:
```JSON
{
"name": "My Gallery",
"images": [
{
"filename": "image.jpg"
}
]
}
```
The `.runwayconf` pre-extension is not required when using the inline `runway-webpack-loader!` loader directive. However, Webpack can also be directed, in the `webpack.config.js` file, to use the `runway-webpack-loader` for any files with a `.runwayconf` pre-extension, such that the import could instead be just
```JavaScript
import runwayConfig from './gallery.runwayconf.json'
```
(see [`runway-webpack-loader`](../runway-webpack-loader/README.md))
### Input
A `runway-react` component receives configuration for the component itself, defining details such as whether the gallery should render images in an overlay when clicked, as well as the configuration details for the gallery rendered by the component.
#### runway-react configuration
Example:
```JavaScript
import Runway from 'runway-react'
<Runway
lightbox={true}
thumbImageType={(props) => {
return <img src={props.metadata.src} />
}}
lightboxImageType={(props) => {
return <img src={props.metadata.src} />
}}
/>
```
Property | Type | Default | Description
:--------|:----:|:-------:|:-----------
lightbox | boolean | `false` | Show image lightbox on image click.
thumbImageType | component | `ImagePlaceholder` | Optional. Must define image component for the thumbnail gallery based on the `props`. See `props` fields below.
lightboxImageType | component | `ScaledImagePlaceholder` | Optional. Must define image component for the lightbox based on the `props`. See `props` fields below.
`thumbImageType` and `lightboxImageType` components receive `props` with the following fields:
Property | Type | Description
:--------|:----:|:-----------
metadata.src | string | The filename of the image (or path and filename, if applicable)
metadata.width | integer | Width, in pixels, of the image file.
metadata.height | integer | Height, in pixels, of the image file.
width | string | (`thumbImageComponent` only) Width integer with CSS units, representing the exact width the image should be rendered to.
height | string | (`thumbImageComponent` only) Height integer with CSS units, representing the exact height the image should be rendered to.
maxWidth | string | (`lightboxImageComponent` only) Width integer with CSS units to which the image should scale down to fit within. The rendered image's width may be smaller if the scale factor to keep its height within the `maxHeight` parameter requires it.
maxHeight | string | (`lightboxImageComponent` only) Height integer with CSS units to which the image should scale down to fit within. The rendered image's height may be smaller if the scale factor to keep its width within the `maxWidth` parameter requires it.
#### Lightbox configuration
If using the lightbox with a `runway-react` component (i.e. with the `lightbox` property set to true), a configuration object will be passed through to the lightbox component. Documentation of this configuration can be found in the [`react-images` package](https://github.com/rcwbr/react-images). Some fields mentioned there cannot be set as props from the `runway-react` component because it sets them internally. These fields are `onClose`, `images`, `isOpen`, `onClickNext`, `onClickPrev` and `onClose`.
```JavaScript
import Runway from 'runway-react'
<Runway
lightboxConfig = {{
width: '70vw',
height: '70vh',
heightOffset: 120
}}
/>
```
Additionally, `runway-react` components set the `spinner` property of their lightbox components to an empty `div` by default, because the default image placeholder effectively replaces the function of the spinner. The spinner can be overridden as a field of the `lightboxConfig` property, either to an arbitrary component, or back to the `react-images` default spinner via an `undefined` value:
```JavaScript
import Runway from 'runway-react'
<Runway
lightboxConfig = {{
spinner: undefined
}}
/>
```
Property | Type | Default | Description
:--------|:----:|:-------:|:-----------
width | string | `'90vw'` | The maximum width of images rendered in the lightbox. Must include CSS units in string. Should not exceed `90vh`, or buttons will render on top of images.
height | string | `'100vh'` | The maximum height of images rendered in the lightbox, plus the `heightOffset` property.
heightOffset | integer | `90` | The height (in pixels) subtracted from the `height` property to determine the maximum height of lightbox images, accounting for the height of the close button and image count text.
#### Runway configuration
The configuration for the gallery rendered by a `runway-react` component is passed in through the `config` property. Usually, this property should be defined by the output of a Runway loader, rather than by direct assignment at `runway-react` component instantiation.
Example:
```JavaScript
import Runway from 'runway-react'
<Runway config={{
name: 'gallery',
imagesFolder: 'images',
thumbsFolder: 'images/thumbs',
width: 960,
imagesPerRow: 4,
imageMargins: {
horiz: 10,
vert: 10
},
images: []
}} />
```
Normally, this property should be read from a Runway loader, as in this example:
```JavaScript
import Runway from 'runway-react'
import runwayConfig from 'runway-webpack-loader!./gallery.runwayconf.json'
<Runway config={runwayConfig} />
```
See [`runway-gallery`](../runway-gallery) for documentation of how to use this configuration.
### Output
The `Gallery` React component is self-contained within a div with an explicit pixel width defined by the `config` prop's `width` field. The height is not self-constrained.
## Implementation details
The image [lightbox](https://github.com/rcwbr/react-images) is part of a very lightly modified version of [jossmac's react-images](https://github.com/jossmac/react-images). The only change is the addition of an optional `component` field of each obect in the Lightbox component's `images` array, which, if present, is rendered in place of the default `<img>` component in the lightbox. This allows the [`react-element-placeholder`](https://todo) to be rendered in the lightbox while the image files load.
<file_sep># Runway-Gallery
`runway-gallery` pre-processes gallery images for static websites. By processing images during site builds, it generates optimally sized thumbnails to minimize site load time without creating any request-time server load.
## Usage
The `runway-gallery` package exports an object with a single attribute, `default`, with a function as the value. That function has two parameters: a configuration object (see [Configuration](Configuration)), and the path in which the gallery photos should be processed (i.e. the directory containing the `imagesFolder` and `thumbsFolder`). It returns a Promise with a resolution parameter of an object that includes all the configuration object's values, and adds the filenames of the processed thumbnail files.
Example usage:
```JavaScript
var runwayGallery = require('runway-gallery')
var galleryConfig = // configuration options object
runwayGallery.default(
galleryConfig,
'.'
).then(gallery => {
// gallery object includes all values from galleryConfig, and adds processed thumbnail filenames
}
```
### Configuration
Example configuration object:
```JSON
{
"name": "My Gallery",
"imagesFolder": "images",
"thumbsFolder": "images/thumbs",
"width": 960,
"imagesPerRow": 4,
"imageMargins": {
"horiz": 10,
"vert": 10
},
"images": [
{
"filename": "image1.jpg"
}
]
}
```
Property | Type | Default | Description
:--------|:----:|:-------:|:-----------
name | string | `'gallery'` | The name to be rendered labelling the gallery
imagesFolder | string | `'images'` | The path, relative to the file instantiating the `runway-react` component, to the full-size image files listed in the `images` property
thumbsFolder | string | `'images/thumbs'` | The path, relative to the file instantiating the `runway-react` component, to which `runway-gallery` will save the generated thumbnail image files
width | integer | `960`| The width, in pixels, of the gallery component
imagesPerRow | integer | `4` | The number of images to place in each row of the thumbnail gallery
imageMargins | object | `{ horiz: 10, vert: 10 }` | The horizontal and vertical margins, in pixels, around each gallery thumbnail
images | array | `[]` | The list of images to render in the gallery as represented by an object -- see below for the definition of this object
Each object in the Runway configuration `images` consist of the following fields:
Property | Type | Description
:--------|:----:|:-----------
filename | string | The filename of the image -- the image file must exist in the `imagesFolder` directory
caption | string | (Optional) The caption to be rendered with the image in the overlay
Example `images` array:
```JSON
[
{
"filename": "image1.jpg"
},
{
"filename": "iamge2.jpg",
"caption": "This photo is the second image in this gallery"
}
]
```
## Use in a build tool
### Webpack
The [`runway-webpack-loader`](../runway-webpack-loader) package defines a loader that wraps `runway-gallery` for use with Webpack.
### Other build tools
Wrappers may be written for `runway-gallery` so that it can be used as a plugin/loader for other build tools used to generate static sites. Use the [`runway-webpack-loader`](../runway-webpack-loader) package as an example of the requirements of such a wrapper.
<file_sep>import React from 'react'
import ReactDOM from 'react-dom'
import { BrowserRouter, Route } from 'react-router-dom'
import Runway from 'runway-react'
import runwayConfig from 'runway-webpack-loader!./testGallery.runwayconf.json'
class Site extends React.Component {
render () {
return (
<div>
<Route
exact path='/'
component={() => (
<div>
<Runway
lightbox={true}
config={runwayConfig}
/>
</div>
)}
/>
</div>
)
}
}
ReactDOM.render(
<BrowserRouter>
<Site />
</BrowserRouter>,
document.getElementById('app')
)
<file_sep>import fs from 'fs'
import sharp from 'sharp'
import defaultConfig from './galleryConfig.js'
function buildGallery (runwayConf, context, callback) {
// apply default config to gallery config
var galleryConfig = defaultConfig(runwayConf)
context = (context !== '') ? context + '/' : context
galleryConfig.imagesFolderFullPath = context + galleryConfig.imagesFolder
galleryConfig.thumbsFolderFullPath = context + galleryConfig.thumbsFolder
// prepare images folder
try {
fs.mkdirSync(galleryConfig.imagesFolderFullPath)
} catch (err) {
if (err.code !== 'EEXIST') throw err
}
// prepare thumbs folder
try {
fs.mkdirSync(galleryConfig.thumbsFolderFullPath)
} catch (err) {
if (err.code !== 'EEXIST') throw err
}
// read metadata from images
var metadataPromises = []
galleryConfig.images.forEach(image => {
metadataPromises.push(sharp(
galleryConfig.imagesFolderFullPath + '/' + image.filename
).metadata())
})
// resolve metadata promises
Promise.all(metadataPromises).then(metadata => {
// copy image meta into images object
galleryConfig.images.forEach((image, index) => {
// image.metadata = metadata[index]
image.metadata = {}
image.metadata.width = metadata[index].width
image.metadata.height = metadata[index].height
})
// distribute images into rows in gallery object
var gallery = {
rows: []
}
var row = {
height: -1,
width: 0,
images: []
}
galleryConfig.images.forEach((image, index) => {
if (row.height === -1 || image.metadata.height < row.height) {
// scale the running row width total by the new image scale
row.width *= (image.metadata.height / row.height)
row.height = image.metadata.height
}
row.images.push(image)
row.width += image.metadata.width * (row.height / image.metadata.height)
// if row has enough images and enough horizontal pixels, push to gallery
var marginWidth = (row.images.length - 1) * galleryConfig.imageMargins.horiz
if (
row.width + marginWidth >= galleryConfig.width
&& row.images.length >= galleryConfig.imagesPerRow
) {
gallery.rows.push(row)
row = {
height: -1,
width: 0,
images: []
}
}
})
// add the last row if it had fewer images than the imagesPerRow setting
if (row.images.length > 0) {
gallery.rows.push(row)
}
gallery.rows.forEach((row) => {
// images will be scaled to gallery width less the horizontal image margins
const galleryImageWidth = (
galleryConfig.width
- galleryConfig.imageMargins.horiz * (row.images.length - 1)
)
row.images.forEach((image) => {
// scale image height to row height
image.scaleFactor = row.height / image.metadata.height
// scale images to fit in row
image.scaleFactor *= galleryImageWidth / row.width
// round down to prevent row overflow
image.width = Math.floor(image.metadata.width * image.scaleFactor)
image.height = Math.floor(image.metadata.height * image.scaleFactor)
// resize image
sharp(galleryConfig.imagesFolderFullPath + '/' + image.filename)
.resize(image.width, image.height)
.toFile(galleryConfig.thumbsFolderFullPath + '/' + image.filename)
})
})
delete galleryConfig.images
galleryConfig.gallery = gallery
callback(galleryConfig)
})
}
export default (runwayConf, context = '') => {
return new Promise(function (resolve, reject) {
buildGallery(runwayConf, context, (gallery) => {
resolve(gallery)
})
})
}
<file_sep>const imageDiv = (last, imageMargins) => {
const allImageDivs = {
float: 'left',
cursor: 'pointer'
}
const imageDivsExceptLast = {
marginRight: imageMargins.horiz + 'px'
}
return last ?
allImageDivs
: {...imageDivsExceptLast, ...allImageDivs}
}
export default {
imageDiv: imageDiv
}
<file_sep>const rowDiv = (last, imageMargins) => {
const allRowDivs = {
clear: 'both',
overflow: 'auto'
}
const rowDivsExceptLast = {
marginBottom: imageMargins.vert + 'px'
}
return last ?
allRowDivs
: {...rowDivsExceptLast, ...allRowDivs}
}
export default {
rowDiv: rowDiv
}
<file_sep>import React from 'react'
import GalleryImage from './components/GalleryImage/index.jsx'
import styles from './styles.js'
export default class GalleryRow extends React.Component {
render () {
const galleryConfig = this.props.galleryConfig
const rowImages = this.props.row.images.map((image, index) => {
const last = (index === this.props.row.images.length - 1)
return (
<GalleryImage
key = {index}
image = {image}
galleryConfig = {galleryConfig}
openOverlay = {this.props.openOverlay}
imageComponentConfig = {this.props.imageComponentConfig}
last = {last}
/>
)
})
return (
<div style = {
styles.rowDiv(this.props.last, this.props.galleryConfig.imageMargins)
}>
{rowImages}
</div>
)
}
}
| eb79f67ca99ad3fc0ad19580df66f279f4ab4e22 | [
"Markdown",
"JavaScript"
] | 7 | Markdown | rcwbr/runway | 45fccdd38c389186c85f3d892aef6197e2adb7a5 | e74722b8e16d8469742034209cacc8449f807d96 | |
refs/heads/master | <file_sep>Je suis <NAME>. Je suis très contente de faire ce bootcamp!
<file_sep>SET SQL_SAFE_UPDATES = 0;
UPDATE salesperson
SET store = 'MIAMI'
WHERE name = "MARTIN";
UPDATE customers
SET email = "<EMAIL>"
WHERE name = "<NAME>";
UPDATE customers
SET email = "<EMAIL>"
WHERE name = "<NAME>";
UPDATE customers
SET email = "<EMAIL>"
WHERE name = "<NAME>";
UPDATE customers
SET email = "<EMAIL>"
WHERE name = "<NAME>";
<file_sep>/*Who Have Published What At Where?*/
SELECT
a.au_id AS AUTHOR_ID,
a.au_lname AS LAST_NAME,
a.au_fname AS FIRST_NAME,
t.title AS TITLE,
p.pub_name AS PUBLISHER
FROM
authors a,
titleauthor ta,
titles t,
publishers p
WHERE
a.au_id = ta.au_id
AND ta.title_id = t.title_id
AND t.pub_id = p.pub_id;
/*Check*/
SELECT
COUNT(*)
FROM
titleauthor;
/*Who Have Published How Many At Where?*/
SELECT
a.au_id AS AUTHOR_ID,
a.au_lname AS LAST_NAME,
a.au_fname AS FIRST_NAME,
p.pub_name AS PUBLISHER,
COUNT(t.title) AS TITLE_COUNT
FROM
authors a,
titleauthor ta,
titles t,
publishers p
WHERE
a.au_id = ta.au_id
AND ta.title_id = t.title_id
AND t.pub_id = p.pub_id
GROUP BY AUTHOR_ID , LAST_NAME , FIRST_NAME , PUBLISHER;
/*Check*/
SELECT
a.au_id AS AUTHOR_ID,
a.au_lname AS LAST_NAME,
a.au_fname AS FIRST_NAME,
p.pub_name AS PUBLISHER,
COUNT(t.title) AS TITLE_COUNT
FROM
authors a,
titleauthor ta,
titles t,
publishers p
WHERE
a.au_id = ta.au_id
AND ta.title_id = t.title_id
AND t.pub_id = p.pub_id
GROUP BY AUTHOR_ID , LAST_NAME , FIRST_NAME , PUBLISHER;
SELECT
SUM(TITLE_COUNT)
FROM
challenge2;
/*Best Selling Authors*/
SELECT
a.au_id AS AUTHOR_ID,
a.au_lname AS LAST_NAME,
a.au_fname AS FIRST_NAME,
SUM(s.qty) AS TITLES_SOLD
FROM
authors a,
titleauthor ta,
titles t,
sales s
WHERE
a.au_id = ta.au_id
AND ta.title_id = t.title_id
AND t.title_id = s.title_id
GROUP BY AUTHOR_ID , LAST_NAME , FIRST_NAME
ORDER BY TITLES_SOLD DESC
LIMIT 3;
/*Best Selling Authors*/
SELECT
a.au_id AS AUTHOR_ID,
a.au_lname AS LAST_NAME,
a.au_fname AS FIRST_NAME,
IFNULL(SUM(s.qty), 0) AS TITLES_SOLD
FROM
authors a
LEFT JOIN
titleauthor ta ON a.au_id = ta.au_id
LEFT JOIN
titles t ON ta.title_id = t.title_id
LEFT JOIN
sales s ON t.title_id = s.title_id
GROUP BY AUTHOR_ID , LAST_NAME , FIRST_NAME
ORDER BY TITLES_SOLD DESC;
<file_sep>/*Challenge 1 - Step 1*/
SELECT
titleauthor.au_id AS authorID,
titleauthor.title_id AS titleID,
titles.advance * titleauthor.royaltyper / 100 AS advance,
titles.price * sales.qty * titles.royalty / 100 AS sales_royalty
FROM
titleauthor
INNER JOIN
titles ON titleauthor.title_id = titles.title_id
INNER JOIN
sales ON titles.title_id = sales.title_id;
/*GROUP BY authorID, titleID*/
/*Challenge 1 - Step 2*/
SELECT
authorID, titleID, SUM(sales_royalty), advance AS sales_royalty_sum
FROM
(SELECT
titleauthor.au_id AS authorID,
titleauthor.title_id AS titleID,
titles.advance * titleauthor.royaltyper / 100 AS advance,
titles.price * sales.qty * titles.royalty / 100 AS sales_royalty
FROM
titleauthor
INNER JOIN titles ON titleauthor.title_id = titles.title_id
INNER JOIN sales ON titles.title_id = sales.title_id) advance_royalty_list
GROUP BY authorID , titleID;
/*Challenge 1 - Step 3*/
SELECT
authorID,
SUM(advance_sum) + SUM(sales_royalty_sum) AS total_profit
FROM
(SELECT
authorID,
titleID,
SUM(sales_royalty) AS sales_royalty_sum,
advance AS advance_sum
FROM
(SELECT
titleauthor.au_id AS authorID,
titleauthor.title_id AS titleID,
titles.advance * titleauthor.royaltyper / 100 AS advance,
titles.price * sales.qty * titles.royalty / 100 AS sales_royalty
FROM
titleauthor
INNER JOIN titles ON titleauthor.title_id = titles.title_id
INNER JOIN sales ON titles.title_id = sales.title_id) advance_royalty_list
GROUP BY authorID , titleID) advance_royalty_aggregated
GROUP BY authorID
ORDER BY total_profit DESC
LIMIT 3;
/*Challenge 2 - Step 1*/
DROP TABLE advance_royalty_list;
CREATE TEMPORARY TABLE advance_royalty_list
SELECT titleauthor.au_id AS authorID,
titleauthor.title_id AS titleID,
titles.advance * titleauthor.royaltyper / 100 AS advance,
titles.price * sales.qty * titles.royalty / 100 AS sales_royalty
FROM titleauthor
INNER JOIN titles
ON titleauthor.title_id = titles.title_id
INNER JOIN sales
ON titles.title_id = sales.title_id;
/*Challenge 2 - Step 2*/
DROP TABLE advance_royalty_aggregated;
CREATE TEMPORARY TABLE advance_royalty_aggregated
SELECT authorID, titleID, SUM(sales_royalty) AS sales_royalty_sum, SUM(advance) as advance_sum
FROM advance_royalty_list
GROUP BY authorID, titleID;
/*Challenge 2 - Step 3*/
CREATE TEMPORARY TABLE result
SELECT authorID, SUM(advance_sum) + SUM(sales_royalty_sum) AS total_profit
FROM advance_royalty_aggregated
GROUP BY authorID
ORDER BY total_profit DESC
LIMIT 3;
/*Challenge 3*/
CREATE TABLE most_profiting_authors (
au_id VARCHAR(45),
profits FLOAT(2)
);
INSERT INTO most_profiting_authors
SELECT * FROM result<file_sep>DELETE FROM cars WHERE id = 1;
SELECT * FROM cars;<file_sep>[LocalizedFileNames]
[email protected],0
<file_sep>Je suis Ji Na
<file_sep>*********Project Description**********
Teenage forum textual data in a csv file provided from a Mongolian start-up company is translated into english using Google Translate API.
*********Project Goals**********
* Handle csv file to create a dataset
* Create a database with different tables in MySQL
* Request MySQL database via python
* Use Google Translate API to translate long texts
**********Diagram to illustrate**********
[Recieved a csv file [Saved the mongolian text [Fetch the mongolian text [Save the translated text [Join two tables of mongolian text
==> ==> from the database and ==> ==> and its translation to create a new table
with mongolian text] into a database table] translate it using Google Translation API] into a new table] with mongolian and english text with its emoji]
**********Resources**********
CSV file with teenage forum data
Google Translate API
**********Links**********
Trello : https://trello.com/b/cuMbUGnX/module-1-project-kanban
Github : https://github.com/jina2020/dataV2-labs.git (module-1/Module 1 project)<file_sep>USE lab_mysql;
CREATE TABLE IF NOT EXISTS `lab_mysql`.`Customers` (
`id` INT NULL AUTO_INCREMENT,
`idCustomer` VARCHAR(45) NOT NULL,
`name` VARCHAR(45) NOT NULL,
`phone_number` VARCHAR(45) NOT NULL,
`email` VARCHAR(45) NOT NULL,
`adress` VARCHAR(45) NULL,
`city` VARCHAR(45) NULL,
`state` VARCHAR(45) NULL,
`country` VARCHAR(45) NULL,
`zip_code` VARCHAR(45) NULL,
UNIQUE INDEX `idCar_UNIQUE` (`id` ASC),
PRIMARY KEY (`idCustomer`))
ENGINE = InnoDB;
CREATE TABLE IF NOT EXISTS `lab_mysql`.`Cars` (
`id` INT NULL AUTO_INCREMENT,
`idCar` VARCHAR(45) NOT NULL,
`manufacturer` VARCHAR(45) NULL,
`model` VARCHAR(45) NULL,
`year` INT NULL,
`color` VARCHAR(45) NULL,
UNIQUE INDEX `id_UNIQUE` (`id` ASC),
PRIMARY KEY (`idCar`))
ENGINE = InnoDB;
CREATE TABLE IF NOT EXISTS `lab_mysql`.`SalesPerson` (
`id` INT NULL AUTO_INCREMENT,
`SalesPersoncol` VARCHAR(45) NOT NULL,
`name` VARCHAR(45) NOT NULL,
`store` VARCHAR(45) NOT NULL,
UNIQUE INDEX `id_UNIQUE` (`id` ASC),
PRIMARY KEY (`SalesPersoncol`))
ENGINE = InnoDB;
CREATE TABLE IF NOT EXISTS `lab_mysql`.`Invoices` (
`id` INT NULL AUTO_INCREMENT,
`idInvoice` VARCHAR(45) NOT NULL,
`date` DATETIME NOT NULL,
`car` VARCHAR(45) NOT NULL,
`customer` VARCHAR(45) NOT NULL,
`salesperson` VARCHAR(45) NOT NULL,
`Customers_idCustomer` VARCHAR(45) NOT NULL,
`SalesPerson_SalesPersoncol` VARCHAR(45) NOT NULL,
`Cars_idCar` VARCHAR(45) NOT NULL,
PRIMARY KEY (`idInvoice`, `Customers_idCustomer`, `SalesPerson_SalesPersoncol`, `Cars_idCar`),
UNIQUE INDEX `id_UNIQUE` (`id` ASC),
INDEX `fk_Invoices_Customers_idx` (`Customers_idCustomer` ASC),
INDEX `fk_Invoices_SalesPerson1_idx` (`SalesPerson_SalesPersoncol` ASC),
INDEX `fk_Invoices_Cars1_idx` (`Cars_idCar` ASC),
CONSTRAINT `fk_Invoices_Customers`
FOREIGN KEY (`Customers_idCustomer`)
REFERENCES `lab_mysql`.`Customers` (`idCustomer`)
ON DELETE NO ACTION
ON UPDATE NO ACTION,
CONSTRAINT `fk_Invoices_SalesPerson1`
FOREIGN KEY (`SalesPerson_SalesPersoncol`)
REFERENCES `lab_mysql`.`SalesPerson` (`SalesPersoncol`)
ON DELETE NO ACTION
ON UPDATE NO ACTION,
CONSTRAINT `fk_Invoices_Cars1`
FOREIGN KEY (`Cars_idCar`)
REFERENCES `lab_mysql`.`Cars` (`idCar`)
ON DELETE NO ACTION
ON UPDATE NO ACTION)
ENGINE = InnoDB;
| 12b93e8af12a58f71d04eb6c9e35ec94cb25cbb6 | [
"Markdown",
"SQL",
"Text",
"INI"
] | 9 | Markdown | jina2020/dataV2-labs | 8153d5a3d947318be492eb474e9d2150b2031f41 | d3620fae412acb95f702da1b1d27a0762b92d1df | |
refs/heads/master | <repo_name>wnsgur0504/Node.js_workspace<file_sep>/project1014/server.js
/*웹서버 구축하기*/
// 모듈이란? 기능을 모아놓은 자바스크립트 파일
var http = require("http"); // http 내부 모듈
var url = require("url"); //url 분석모듈
var fs = require("fs"); //file system
var mysql = require("mysql");
var ejs = require("ejs"); //node 서버에서만 실행가능한 문서
// html로 채워졌다고 하여, html문서로 보면 안됨.
var port = 7878;
// mysql 접속 문자열
let conStr = {
url:"localhost",
user:"root",
password:"<PASSWORD>",
database:"node"
};
var con; //mysql 접속 정보를 가진 객체, 이 객체로 sql문 수행가능
// 서버 객체 생성
var server = http.createServer(function(req, res){
// 클라이언트가 원하는 요청을 처리하려면, URL을 분석하여 URI추출해서 조건을 따져보자
var urlJson = url.parse(req.url, true); //분석결과를 json으로 반환해줌
console.log("URL 분석 결과는 ", urlJson);
res.writeHead(200, {"Content-Type":"text/html; charset=utf-8"});
if(urlJson.pathname=="/"){
fs.readFile("./index.html", "utf-8", function(error, data){
if(error){
console.log("index 파일 읽기 에러 :" ,error);
}else{
res.end(data);
}
});
}else if(urlJson.pathname=="/member/registForm"){
fs.readFile("./registForm.html", "utf-8", function(error, data){
if(error){
console.log("registForm 읽기 에러 :" ,error);
}else{
res.end(data);
}
});
}else if(urlJson.pathname=="/member/loginForm"){
fs.readFile("./loginForm.html", "utf-8", function(error, data){
if(error){
console.log("loginForm 읽기 에러 :" ,error);
}else{
res.end(data);
}
});
}else if(urlJson.pathname=="/member/regist"){
// 브라우저에서 전송된 파라미터를 먼저 받아야 된다.
// get방식의 파라미터 받기
// 회원정보는 member2 테이블에 넣고
var params = urlJson.query;
var sql = "insert into member2(uid, password, uname, phone, email, receive, addr, memo)";
sql += " values(?, ?, ?, ?, ?, ?, ? ,?)"; //물음표는 바인드 변수를 의미
con.query(sql,
[params.uid,
params.password,
params.uname,
params.phone,
params.email,
params.receive,
params.addr,
params.memo], function(error, result, fields){
if(error){
console.log("회원정보 insert 실패 : ", error);
}else{
// mysql에서 지원하는 last_insert_id()를 조회해서
// 방금 insert된 회원의 pk를 조회해보자
sql = "select last_insert_id() as member2_id";
con.query(sql, function(error, record, fields){
if(error){
console.log("pk가져오기 실패 : ", error);
}else{
// member2 정보가 insert 성공 시
// 스킬정보는 member_skill에 넣자(배열 길이만큼)
// console.log("record : ", record);
var member2_id = record[0].member2_id;
for(var i=0;i<params.skill_id.length;i++){
sql = "insert into member_skill(member2_id, skill_id) values("+member2_id+","+params.skill_id[i]+")";
// console.log("스킬 등록 쿼리 : ", sql);
// 쿼리 실행
con.query(sql, function(err){
if(err){
console.log("회원정보 등록 실패");
}else{
res.end("회원정보 등록 완료")
}
});
}
}
}); //select 쿼리문 수행
}
});
}else if(urlJson.pathname=="/member/list"){
// 회원목록보여주기
var sql = "select * from member2";
con.query(sql, function(error, record, fields){
if(error){
}else{
// console.log("회원목록 : ", record);
// 테이블로 구성하여 출력
var tag = "<table width='100%' border='1px'>";
tag +="<tr>";
tag +="<th>member2_id</th>";
tag +="<th>uid</th>";
tag +="<th>password</th>";
tag +="<th>uname</th>";
tag +="<th>phone</th>";
tag +="<th>email</th>";
tag +="<th>receive</th>";
tag +="<th>addr</th>";
tag +="<th>memo</th>";
tag +="</tr>";
for(var i=0;i<record.length;i++){
tag +="<tr>";
tag +="<td><a href='/member/detail?member2_id="+record[i].member2_id+"'>"+record[i].member2_id+"</a></td>"
tag +="<td>"+record[i].uid+"</td>"
tag +="<td>"+record[i].password+"</td>"
tag +="<td>"+record[i].uname+"</td>"
tag +="<td>"+record[i].phone+"</td>"
tag +="<td>"+record[i].email+"</td>"
tag +="<td>"+record[i].receive+"</td>"
tag +="<td>"+record[i].addr+"</td>"
tag +="<td>"+record[i].memo+"</td>"
tag +="</tr>";
}
tag += "<tr>";
tag += "<td colspan='9'><a href='/'>메인으로</a></td>";
tag += "</tr>";
tag += "</table>";
res.end(tag);
}
});
}else if(urlJson.pathname=="/member/detail"){ //회원의 상세정보
var member2_id = urlJson.query.member2_id;
var sql = "select * from member2 where member2_id="+member2_id;
con.query(sql ,function(error, record, fields){
if(error){
console.log("회원 상세조회 실패 : ", error);
}else{
var member = record[0];
fs.readFile("./detail.ejs", "utf-8", function(error, data){
if(error){
console.log("detail.html 읽기 실패 : ", error);
}else{
res.end(ejs.render(data, { //detail.ejs에게 json형태의 데이터(변수에 담긴)를 보낸다
uid:member.uid,
uname:member.uname,
phone:member.phone,
addr:member.addr,
memo:member.memo
}));
}
});
}
});
}else if(urlJson.pathname=="/fruit"){
var f1 = "apple";
var f2 = "banana";
fs.readFile("./test.ejs", "utf-8", function(error, data){
// ejs를 그냥 파일로 문자취급해서 보내면 원본 코드까지 가버리기
// 때문에, 서버에서 실행은 한 후, 그 결과를 보내야한다..(jsp, php, asp의 원리)
res.end(ejs.render(data,{
"fruit":f1
}));
});
}
// 200이란?? HTTP 상태코드
// 404 - file not found
// 500 - critical error
});
function getConnection(){
con = mysql.createConnection(conStr); //DBMS정보인 json 매개변수
}
// 서버가동
server.listen(port, function(){
console.log("Server is running at "+port+"...");
getConnection();
});<file_sep>/project1012/server4.js
/*----------------------------------------
MySQL 연동 해보자
----------------------------------------*/
var mysql = require("mysql");
// 접속에 필요한 정보
let conStr = {
url:"localhost",
user:"root",
password:"<PASSWORD>",
database:"node"
}
//접속시도 후, 접속정보가 반환된다..
var con = mysql.createConnection(conStr);
//반환된 con을 이용하여 쿼리문 수행
var sql = "insert into member(firstname, lastname, local, msg)";
sql += "values ('준혁', '최', 'Seoul', '하이하이 ^^;')";
con.query(sql, function(error, result, fields){
if(error){
console.log("등록실패;");
}else{
console.log("등록성공!");
}
}); //쿼리실행
<file_sep>/project1012/module.js
/*
Node.js가 전세계적으로 열풍을 일으킨 이유는?
가장 큰 이유가, 바로 모듈때문이다!
모듈이란? 우리가 지금까지 자바스크립트 라이브러리를, 파일로 저장해놓은 단위
node.js모듈의 특징 : 전세계 많은 개발자들이 각자 자신이 개발한 모듈을 공유하고 있다.
따라서 지금 이순간에도 새로운 모듈이 계속 추가되고 있다..
[모듈의 유형]
1) 내장 모듈
- os 모듈
- url 모듈
- file system 모듈 (★★★)
2) 사용자 정의 모듈
*/
// os 모듈
// 자바스크립트와는 달리, 모듈을 가져올 때는 require()함수를 이용해야한다..
// var os = require("os"); //이미 내장된 모듈중 os모듈을 가져오기
// console.log(os.hostname()); //컴퓨터 이름
// console.log(os.cpus()); //cpu
// url 모듈 : url정보를 분석해주는 모듈
// var url = require("url");
// // url을 분석하여, 그 결과를 result변수에 담아보자!
// var result = url.parse("https://terms.naver.com/search.nhn?query=apple")
// console.log("검색어는 ",result.query);
// file system 모듈 : javascript에선 불가능했던 파일접근을 가능케 해주는 모듈
// 로컬상의 파일을 읽어오거나, 쓸수 있는 모듈
var fs = require("fs");
// 지정한 경로의 파일을 읽어서, 다 읽혀지면, 두번째 인수인익명함수를
// 호출한다. 이렇게 특정 이벤트가 발생할 때, 시스템에 의해 역으로 호출되는 유형의 함수를
// Callback함수라 한다!!!
//callback함수의 첫번째 매개변수 (error) :에러 정보를 담고 있는 객체 반환
//callback함수의 두번째 매개변수(data) : 실제 읽어들인 파일의 내용을 담고 있다..
fs.readFile("./data/memo.txt", "utf-8",function(error, data){
console.log("파일을 모두 읽기 완료!");
console.log(data);
});<file_sep>/project1012/server3.js
/*
Node.js에서 오라클과 연동해보자!!
*/
// 오라클을 접속하려면, 접속을 담당하는 모듈을 사용해야한다.
// 우리가 node.js를 설치하면 아주 기본적인 모듈만 내장되어 있기 때문에
// 개발에 필요한 모듈은 그때 그때 다운으로 받아 설치해야한다!! (인기비결)
var oracledb = require("oracledb");
// 변경할 목적의 데이터가 아니라면, 상수로 선언하자!
let conStr = {
user:"user0907",
password:"<PASSWORD>",
connectionString:"localhost/XE"
}; //오라클에 접속할 때 필요한 정보!!
// 오라클에 접속을 시도해본다!!
// 콜백함수
oracledb.getConnection(conStr, function(error, con){
if(error){ //실패 시
console.log("접속 실패 : ", error);
}else{
// 테이블에 데이터를 넣어보자!
// 접속객체를 이용하여 insert쿼리를 날려보자!
console.log("접속 성공");
insert(con);
}
});
function insert(con){
var sql = "insert into member2(member2_id, firstname, lastname, local, msg)";
sql += "values(seq_member2.nextval, 'JunHyuk', 'Choi', 'America', 'Hello ORACLE!')";
// 쿼리 실행
con.execute(sql, function(error, result, fields){
if(error){
console.log("삽입 실패 : ", error);
}else{
console.log("삽입 성공");
}
});
}<file_sep>/project1012/test.js
/*
node.js의 저장형식은 당연히 확장자가 .js이다.
node.js는 html문서를 제어했던 예전의 js와는 목적이 다르므로,
실행또한 html문서에서 하는 것이 아니라, 단독 실행하면 된다.
따라서 test.js 파일이 있는 곳으로 가서, node 파일명 으로 실행하면 된다.
*/
console.log("Hello JS World!");<file_sep>/project1014/server3.js
/*
ejs를 이해해보자
ejs란?
- 오직, Node.js 서버측에서 해석 및 실행될 수 있는 파일
- js의 문법 사용이 가능 (if, for, 변수선언....)
- 다른 서버 측 스크립트 언어인 PHP, ASP, JSP와 같은 목적
*/
var http = require("http");
var fs = require("fs");
var ejs = require("ejs");
var server = http.createServer(function(req, res){
fs.readFile("./list.ejs", "utf-8", function(error, data){
if(error){
console.log("list 파일 읽기 에러 : ", error);
}else{
res.writeHead(200, {"Content-Type":"text/html; charset=utf8"});
res.end(ejs.render(data));
}
});
});
server.listen(7979, function(){
console.log("Node Server is running port at 7979...");
});
<file_sep>/project1014/server2.js
var http = require("http");
var url = require("url");
// var body = require("body");
var querystring = require("querystring"); //get뿐만아니라 post까지 파싱가능
var server = http.createServer(function(req, res){
// console.log("클라이언트 요청 방식 : ", req.method);
var method = req.method;
if(method=="GET"){
var urlJson = url.parse(req.url, true);
var paramJson = urlJson.query;
res.writeHead(200, {"Content-Type":"text/html; charset=utf-8"});
res.end("클라이언트가 GET방식으로 요청했네요.<br> ID : "+paramJson.id+"<br> Pass : "+paramJson.pass);
// console.log("GET URL 분석 : ", urlJson);
// console.log("ID : ", paramJson.id);
// console.log("Pass : ", paramJson.pass);
}else if(method=="POST"){
// Post방식으로 전달된 데이터를 받기 위한 이벤트를 감지해보자!!
res.writeHead(200, {"Content-Type":"text/html; charset=utf-8"});
res.end("클라이언트가 POST방식으로 요청했네요");
// body로 전송된 데이터는 url 분석(parse)만으로는 해결이 안된다!
// var urlJson = querystring.parse(req.url);
req.on("data", function(param){
// var postParam = url.parse(new String(param).toString(), true);
var postParam = querystring.parse(new String(param).toString());
console.log("POST 전송된 파라미터는 : ", postParam);
console.log("ID : "+postParam.id+" PW : " +postParam.pass);
});
}
});
server.listen(9999, function(){
console.log("My Server is running at 9999....");
});
<file_sep>/project1013/server.js
/*
http 웹서버 구축하기
*/
var http = require("http"); //필요한 모듈 가져오기
var fs = require("fs");
var url = require("url"); //url 정보를 해석해주는 모듈
var mysql = require("mysql"); //외부모듈이기 때문에, npm install 설치 필요
var port = 8888;
var con; //접속 정보를 가진 객체를 받는 전역 변수
// 서버객체 생성
// request, response는 이미 Node.js 자체적으로 존재하는 객체
var server = http.createServer(function(req, res){
// 클라이언트의 요청에 대한 응답처리... (html 문서를 주고 받고 있음)
// 클라이언트의 요청 내용이 다양하므로, 각 요청을 처리할 조건문이 있어야 한다..
// 따라서, 클라이언트가 원하는 것이 무엇인지부터 파악!
console.log("클라이언트의 요청 url : ", req.url);
// url 모듈을 이용하여 전체 주소를 대상으로 해석시작
// 파싱 시, true 옵션을 주면 파라미터들을 JSON형태로 준다.
var result = url.parse(req.url, true);
var params = result.query; //파라미터를 담고 있는 json객체반환
res.writeHead(200, {"Content-Type":"text/html; charset=utf-8"});
if(result.pathname=="/login"){
console.log("mysql 연동하여 회원 존재여부 확인");
// mysql 연동
var sql = "select * from hclass where id='"+params.m_id+"' and pass='"+params.m_pw+"'";
// console.log(sql);
con.query(sql, function(error, record, fields){
if(error){
console.log("쿼리 실행 실패", error);
}else{
// console.log("record : ", record);
if(record.length==0){
// 로그인 실패 시
console.log("회원 인증실패");
res.end("<script>alert('회원정보가 일치하지 않습니다.'); history.back();</script>");
}else{
// 로그인 성공 시
console.log("회원 인증성공");
res.end("<script>alert('로그인을 성공하셨습니다.')</script>");
}
}
});
// var sql = "select * from hclass where id='파라미터ID값' and pass='<PASSWORD>'";
}else if(result.pathname=="/board"){
console.log("게시판 화면");
res.end("미구현..");
}else if(result.pathname=="/loginForm"){
// 우리가 제작한 loginForm.html은 로컬 파일로 열면 안되고,
// 모든 클라이언트가 인터넷 상의 주소로 접근하게 하기 위해서
// 서버가 html내용을 읽고, 그 내용을 클라이언트로 보내야 한다.
fs.readFile("./loginForm.html", "utf-8", function(error, data){
if(error){
console.log("error 발생!/",error);
}else{
// 읽기 성공이므로, 클라이언트의 응답정보로 보내자
// HTTP 프로토콜로 데이터를 주고 받을때는, 이미 정해진 규약이 있으므로 눈에 보이지않는
// 수많은 설정 정보값들을 서버와 클라이언트간 교환한다
res.end(data);
}
})
}
// 전체 url중에서도 uri만 추출해보자!
// 따라서, 전체 url을 해석해야한다.. 해석은 parsing한다고 한다.
});
// mysql 접속
function connectDB(){
con = mysql.createConnection({
url:"localhost",
user:"root",
password:"<PASSWORD>",
database:"node"
});
}
// 서버가동
server.listen(port, function(){
console.log("Server is running at 8888...");
connectDB(); //웹서버가 가동되면, mysql을 접속해놓자
});
<file_sep>/project1013/server2.js
// 웹 서버 구축
var http = require("http");
var mysql = require("mysql");
const { parse } = require("path");
var url = require("url");
var fs = require("fs");
var con; //mysql 접속 성공 시 그 정보를 보유한 객체. 해당 객체가 있어야 접속된 상태에서
// 쿼리문을 수행할 수 있다.
var port = 9999;
var server = http.createServer(function(req, res){
res.writeHead(200, {"Content-Type":"text/html; charset=utf8"});
// 클라이언트가 원하는 것이 무엇인지를 구분하기 위한 url분석
console.log("URL : ", req.url); //한줄로 되어 있어서, 구분을 못함
// 섞여있는 url을 parsing하기 위해서 전담 모듈을 이용해서 uri와 parameter를 분리
// 분석(파싱) 시 true를 매개변수로 전달하면, 파라미터들을 {Json}으로 묶어준다.
// 장점? 다루기 쉬워짐 (객체 표기법), 배열보다 낫다
var parseObj = url.parse(req.url, true);
console.log(parseObj);
// console.log("URL분석결과 =>", parseObj);
if(parseObj.pathname=="/member/registForm"){
fs.readFile("./registForm.html","utf-8", function(error, data){
if(error){
console.log("파일 읽기 에러 : ", error);
}else{
res.end(data);
}
});
}else if(parseObj.pathname=="/member/regist"){ //회원가입을 원하면
// mysql에 insert할 예정
// 클라이언트의 브라우저에서 전송되어온 파라미터(변수)들을 받아보자!
var params = parseObj.query;
var sql="insert into member2(uid,password,uname,phone,email,receive,addr,memo)";
sql+=" values(?,?,?,?,?,?,?,?)"; //바인드 변수를 이용
con.query(sql,[params.uid, params.password, params.uname,
params.phone, params.email, params.receive, params.addr,
params.memo]
, function(error, result, fields){
if(error){
console.log("등록 실패 : ", error);
}else{
var msg="<script>";
msg += "location.href='/member/list';";
// /member/list 로 재접속 (클라이언트가 지정한 주소로 재접속함)
msg +="</script>";
res.end(msg);
}
});
}else if(parseObj.pathname=="/member/list"){ //회원목록을 원하면
// mysql에 select할 예정
var sql = "select * from member2";
var tag;
con.query(sql, function(error, record, fields){
if(error){
console.log("가져오기 실패 : ", error);
}else{
tag="<table border='1px' width='80%'>";
tag+="<tr>";
tag+="<th>member2_id</th>";
tag+="<th>uid</th>";
tag+="<th>password</th>";
tag+="<th>uname</th>";
tag+="<th>phone</th>";
tag+="<th>email</th>";
tag+="<th>receive</th>";
tag+="<th>addr</th>";
tag+="<th>memo</th>";
tag+="</tr>";
for(var i=0;i<record.length;i++){
tag+="<tr>";
tag+="<td>"+record[i].member2_id+"</td>";
tag+="<td>"+record[i].uid+"</td>";
tag+="<td>"+record[i].password+"</td>";
tag+="<td>"+record[i].uname+"</td>";
tag+="<td>"+record[i].phone+"</td>";
tag+="<td>"+record[i].email+"</td>";
tag+="<td>"+record[i].receive+"</td>";
tag+="<td>"+record[i].addr+"</td>";
tag+="<td>"+record[i].memo+"</td>";
tag+="</tr>";
}
tag+="</table>";
res.end(tag);
}
});
}else if(parseObj.pathname=="/member/del"){ //회원탈퇴
res.end("회원삭제");
}else if(parseObj.pathname=="/member/edit"){ //회원수정
res.end("회원수정");
}
});
// mysql 접속
function connectDB(){
con = mysql.createConnection({
url:"localhost",
user:"root",
password:"<PASSWORD>",
database:"node"
});
}
server.listen(port, function(){
console.log("Web Server is running at port "+port);
connectDB();
});
<file_sep>/project1015/server.js
var http = require("http");
var url = require("url");
var fs = require("fs");
var ejs = require("ejs");
var mysql = require("mysql");
var qs = require("querystring");
const { uptime } = require("process");
let con; //mysql connection객체
var urlJson;
var server =http.createServer(function(req, res){
// 요청 구분
urlJson=url.parse(req.url, true);
console.log(urlJson);
if(urlJson.pathname=="/"){//"메인을 요청하면"
fs.readFile("./index.html","utf-8", function(error, data){
if(error){
console.log("index 파일 읽기 실패");
}else{
res.writeHead(200, {"Content-Type":"text/html; charset=utf8"});
res.end(data);
}
});
}else if(urlJson.pathname=="/member/registForm"){//가입폼을 요청하면
registForm(req ,res);
}else if(urlJson.pathname=="/member/regist"){ // 가입을 요청하면..
regist(req ,res);
}else if(urlJson.pathname=="/member/loginForm"){ // 로그인 폼을 요청하면..
}else if(urlJson.pathname=="/member/list"){ //회원 목록을 요청하면
getList(req ,res);
}else if(urlJson.pathname=="/member/detail"){//회원정보 보기를 요청하면..
getDetail(req ,res);
}else if(urlJson.pathname=="/member/del"){ //회원정보 삭제를 요청
del(req, res);
}else if(urlJson.pathname=="/member/edit"){//회원정보 수정를 요청
update(req, res);
}else if(urlJson.pathname=="/category"){ //동물구분을 요청하면
getCategory(req, res);
}else if(urlJson.pathname="/animal"){
getAnimal(req, res);
}
});
// 데이터베이스 연동인 경우엔 함수에 별도로 정의
function registForm(req ,res){
// 회원가입폼은 디자인을 표한하기 위한 파일이므로, 기존에는 html로 충분했으나..
// 보유기술은 DB의 데이터를 가져와서 반영해야하므로, ejs로 처리해야함..
var sql = "select * from skill";
con.query(sql, function(error, record, fields){
if(error){
console.log("skill 조회 실패:", error);
}else{
// console.log("skill record : ", record);
// registForm.ejs에게 json배열을 전달하자
fs.readFile("./registForm.ejs", "utf-8", function(error, data){
if(error){
console.log("registForm 파일 읽기 실패 : ", error);
}else{
res.writeHead(200, {"Content-Type":"text/html; charset=utf8"});
res.end(ejs.render(data, {
skillArray:record
}));
}
});
}
});
}
// 회원등록 처리
function regist(req, res){
// post방식으로 전송된, 파라미터받기!!
// body에 있는 파라미터받기
// url모듈에게 파싱을 처리하게 하지말고, querystring 모듈로 처리한다.
req.on("data", function(param){
// console.log(new String(param).toString()); //알아볼수 없는 인코딩 데이터로 넘어옴 -> 문자열화
// 디코드는 성공했지만 파싱이 안되었음 -> querystring 쓰는 이유
var postParam = qs.parse(new String(param).toString());
var sql = "insert into member2(uid, <PASSWORD>, uname, phone, email, receive, addr, memo)";
sql += " values(?, ?, ?, ?, ?, ?, ?, ?)";
con.query(sql,
[postParam.uid
, postParam.password
, postParam.uname
, postParam.phone
, postParam.email
, postParam.receive
, postParam.addr
, postParam.memo], function(error, fields){
if(error){
console.log("등록실패", error);
}else{
// 목록페이지 보여주기
// 등록되었음을 alert()으로 알려주고, /member/list로 재접속
res.writeHead(200, {"Content-Type":"text/html; charset=utf8"});
var tag = "<script>";
tag += "alert('등록성공');";
tag += "location.href='/member/list'";
tag += "</script>";
res.end(tag);
}
});
});
}
function getList(req, res){
// 회원목록 가져오기
var sql = "select * from member2";
con.query(sql, function(error, record, fields){
fs.readFile("./list.ejs", "utf8", function(error, data){
if(error){
console.log("list 파일읽기실패 : ", error);
}else{
res.writeHead(200,{"Content-Type":"text/html; charset=utf8"});
res.end(ejs.render(data,{
memberArray:record
}));
}
})
})
}
function getDetail(req ,res){
// var urlJson = url.parse(req.url, true);
// console.log(urlJson.query.member2_id);
var sql = "select * from member2 where member2_id="+urlJson.query.member2_id;
con.query(sql, function(error, record, fields){
if(error){
console.log("member2 조회 실패 : ", error);
}else{
console.log("record : ",record);
fs.readFile("./detail.ejs", "utf-8", function(error, data){
if(error){
console.log("detail 파일열기 실패 : ", error);
}else{
res.writeHead(200,{"Content-Type":"text/html; charset=utf8"});
res.end(ejs.render(data, {
member:record[0]
}));
}
});
}
});
}
//회원 1명 삭제
function del(req, res){
// get 방식으로 전달된 파라미터 받기
var member2_id = urlJson.query.member2_id;
var sql = "delete from member2 where member2_id="+member2_id;
//error, fields : DML (insert, update, delete)
//error, record, fields : select
con.query(sql, function(error, fields){
if(error){
console.log("삭제 실패 : ", error);
}else{
// alert 띄우고, 회원 목록 보여주기
res.writeHead(200, {"Content-Type":"text/html; charset=utf-8"});
var tag = "<script>";
tag += "alert('회원탈퇴 완료!');";
tag += "location.href='/member/list'";
tag += "</script>"
res.end(tag);
}
});
}
// 회원정보 수정처리
function update(req, res){
// post로 전송된 파라미터들을 받자!!
req.on("data", function(param){
// console.log(param); 인코딩된 상태의 파라미터들
//스트링으로 디코딩하고 querystring으로 파싱
var postParam = qs.parse(new String(param).toString());
var sql = "update member2 set phone=?, email=?, addr=?, memo=?";
sql += ", password=?, receive=? where member2_id=?";
con.query(sql, [
postParam.phone,
postParam.email,
postParam.addr,
postParam.memo,
postParam.password,
postParam.receive,
postParam.member2_id
], function(error, fields){
if(error){
console.log("수정 실패 : ", error);
}else{
res.writeHead(200, {"Content-Type":"text/html; charset=utf-8"});
var tag = "<script>";
tag += "alert('회원정보 수정완료!');";
tag += "location.href='/member/detail?member2_id="+postParam.member2_id+"';";
tag += "</script>"
res.end(tag);
}
});
// console.log(postParam);
// 검증용
// var sql = "update member2 set phone='"+postParam.phone+"', email='"+postParam.email+"', memo='"+postParam.memo+"'";
// sql += ", password='"+postParam.password+"', receive='"+postParam.receive+"' where member2_id=?";
});
}
// 카테고리의 종류 가져오기
function getCategory(req, res){
var sql = "select * from category";
con.query(sql, function(error, record, fields){
if(error){
console.log("동물구분 목록 조회실패", error );
}else{
fs.readFile("./animal.ejs", "utf-8", function(err, data){
if(err){
console.log("animal.ejs 파일 읽기 실패", err);
}else{
res.writeHead(200, {"Content-Type":"text/html; charset=utf-8"});
res.end(ejs.render(data, {
categoryArray:record
}));
}
});
}
});
}
// 동물의 목록 가져오기
function getAnimal(req, res){
var sql = "select * from category";
con.query(sql, function(error, record, fields){
if(error){
console.log("동물구분 목록 조회실패 ", error);
}else{
var categoryRecord=record; //카테고리 목록 배열
var category_id = urlJson.query.category_id; // get방식의 category_id 파라미터 받기
sql = "select * from animal where category_id="+category_id;
// mysql 연동
con.query(sql, function(error, record, fields){
if(error){
console.log("동물목록 가져오기 실패 ", error);
}else{
console.log("record : ", record);
fs.readFile("./animal.ejs", "utf-8", function(err, data){
if(err){
console.log("animal.ejs 가져오기 실패", err);
}else{
res.writeHead(200, {"Content-Type":"text/html; charset=utf-8"});
res.end(ejs.render(data, {
animalArray:record,
categoryArray:categoryRecord,
category_id:category_id
}));
}
});
}
});
}
});
}
// mysql 접속
function connect(){
con = mysql.createConnection({
url:"localhost",
user:"root",
password:"<PASSWORD>",
database:"node"
});
}
server.listen(7788, function(){
console.log("My Server is running at 7788...");
connect();
});<file_sep>/project1012/server.js
/*
HTTP 모듈을 이용하면, 단 몇줄만으로도 웹서버를 구축할 수 있다!
*/
var http = require("http"); //모듈 가져오기!
// http 모듈안에서 지원하는 서버 객체 생성
var server = http.createServer();
// 접속자가 들어오는지 확인해본다
server.on("connection", function(){
console.log("접속 발생");
});
// 서버 가동(포트번호 지정과 함께)
server.listen(8989, function(){
console.log("My Server is running at 8989!");
});
<file_sep>/project1012/server5.js
/*
웹서버를 구축하여, 클라이언트가 전송한 파라마터 값들을
mysql에 넣어보자
*/
var http = require("http"); //http 모듈 가져오기
var fs = require("fs"); //file system module
let port = 7979;
// http 모듈로부터 서버 객체 생성하기
var server = http.createServer(function(req, res){
// 아래의 코드들이 동작하기 전에, 서버는 클라이언트가 원하는게 무엇인지 구분을 먼저 해야된다.
// 요청분석!
// 요청분석을 들어가려면, 요청 정보를 담고 있는 request 객체를 이용해야한다!!
console.log(req.url);
// 1)클라이언트가 회원가입 양식을 보길 원하면 아래의 코드가 수행...
// 서버에 존재하는 회원가입양식 폼파일을 읽어서, 클라이언트에 보내주자
fs.readFile("./회원가입양식.html", "utf-8", function(error, data){
if(error){
console.log("에러 발생 : ", error);
}else{
console.log("파일 읽기 성공!");
// http 프로토콜의 약속 상 형식을 지켜서 보내야함
// 상태코드에 대한 응답헤더를 지정
res.writeHead(200, {"Content-Type":"text/html; charset=utf-8"});
res.end(data);
}
});
//2) 가입을 원할 경우엔, db에 넣어줘야하고
//3) 이미지를 원하면 이미지 보여줘야하고
//4) 오디오파일을 원하면 오디오파일을 전송.....
});
//서버가동하기
server.listen(port, function(){
console.log("Server is running at "+port+"..");
});
| e0f05cac7b158edbab3d4f78b6bd9d44911ccc35 | [
"JavaScript"
] | 12 | JavaScript | wnsgur0504/Node.js_workspace | 902686e1da31affc30e1f8b469a80d1d5e66a969 | c1de3f99ac607214f7cbc1605eb9f0c25fb89afa | |
refs/heads/master | <repo_name>william-marquetti/Exercicios_Java_Orientacao_Objeto3<file_sep>/Exercicios_Java_Orientacao_Objeto3/src/exercicios2/ComparacaoImpl.java
package exercicios2;
import java.util.List;
public class ComparacaoImpl implements IComparacao{
@Override
public void buscaProdutoNomeCodBarras(List<Produto> vetorProdutos, String produtoPesquisado) {
int tamanhoVetor = vetorProdutos.size();
int posicao = 0;
boolean encontrado = false;
System.out.println("\n---------------------------------------");
System.out.println("Comparador de Produtos");
System.out.println("---------------------------------------");
for (Produto produtos : vetorProdutos) {
posicao++;
if ( (produtos.getNome().equals(produtoPesquisado) || produtos.getCodigoBarras().equals(produtoPesquisado))){
System.out.println("\nProduto encontrado. Posição: "+ (posicao-1));
System.out.println("---------------------------------------");
System.out.println(produtos.toString());
encontrado = true;
}else if ( (posicao == tamanhoVetor) && !encontrado) {
System.out.println("Produto não encontrado");
}
}
}
}
<file_sep>/Exercicios_Java_Orientacao_Objeto3/src/exercicio1/Conta_Corrente.java
package exercicio1;
import java.text.DecimalFormat;
public class Conta_Corrente {
DecimalFormat df = new DecimalFormat("#0.00");
private String numero;
private String agencia;
private String cliente;
private String banco;
private double saldo;
public Conta_Corrente(String numero, String agencia, String cliente, String banco, double saldo){
this.numero = numero;
this.agencia = agencia;
this.cliente = cliente;
this.banco = banco;
this.saldo = saldo;
}
public String getNumero() {
return numero;
}
public void setNumero(String numero) {
this.numero = numero;
}
public String getAgencia() {
return agencia;
}
public void setAgencia(String agencia) {
this.agencia = agencia;
}
public String getCliente() {
return cliente;
}
public void setCliente(String cliente) {
this.cliente = cliente;
}
public String getBanco() {
return banco;
}
public void setBanco(String banco) {
this.banco = banco;
}
public double getSaldo() {
return saldo;
}
public void setSaldo(double saldo) {
this.saldo = saldo;
}
public void sacar(double valor){
this.saldo = saldo - (valor + (valor * 0.005));
}
public void depositar(double valor){
this.saldo = saldo +valor;
}
public void extrato(){
String extrato;
extrato = "\nCliente: "+ this.getCliente();
extrato += "\nBanco: "+ this.getBanco();
extrato += "\nConta: "+ this.getNumero() + " Agência: "+ this.getAgencia();
extrato += "\nSaldo: R$"+ df.format(this.getSaldo());
System.out.println(extrato);
}
}
<file_sep>/Exercicios_Java_Orientacao_Objeto3/src/exercicios2/IComparacao.java
package exercicios2;
import java.util.List;
public interface IComparacao {
/**
* Método criado para realizar pesquisa de produtos pelo nome.
*
* Foi utilizado como "static" porque a classe loja não é uma classe de objeto,
* portanto ela não pode ser instanciada.
* Ao definir o método como "static", o método passa a existir sem ter a necessidade
* de instaciar um objeto da classe.
* Esta é a única maneira de acessar um método de uma classe que não pode ser
* instaciada.
*
* @param vetorProdutos é a lista de produtos (ArrayList)
* @param produtoPesquisado é o termo (item, nome, etc) pesquisado (String)
*
* O método buscaProduto, realizará buscas na lista (ArrayList) de produtos,
* retornando as informações do produto, se encontrado.
* A pesaquisa é feita através do método "equals()", onde o termo pesquisado
* só retornará os dados nos casos em que o nome seja EXATAMENTE igual ao termo da
* pesquisa.
*/
public abstract void buscaProdutoNomeCodBarras(List<Produto> vetorProdutos, String produtoPesquisado);
}
<file_sep>/Exercicios_Java_Orientacao_Objeto3/src/exercicio1/Teste.java
package exercicio1;
public class Teste {
public static void main(String[] args) {
Cliente_Especial cc2 = new Cliente_Especial("1515", "1221", "Andiara", "Banco do Brasil", 1500d);
Conta_Corrente cc1 = new Conta_Corrente("0021212", "1313", "William", "Caixa", 1500d);
cc1.extrato();
cc2.extrato();
cc1.sacar(100);
cc2.sacar(100);
cc1.extrato();
cc2.extrato();
}
}
<file_sep>/Exercicios_Java_Orientacao_Objeto3/src/exercicios3/Retangulo.java
package exercicios3;
import java.text.DecimalFormat;
public class Retangulo extends Formas {
DecimalFormat df = new DecimalFormat("#0.00");
private double base;
private double altura;
public Retangulo(){
}
public Retangulo(double altura, double base) {
setBase(base);
setAltura(altura);
calculaArea();
calculaPerimetro();
}
public double getBase() {
return base;
}
public void setBase(double base) {
this.base = base;
}
public double getAltura() {
return altura;
}
public void setAltura(double altura) {
this.altura = altura;
}
@Override
public void calculaPerimetro() {
setPerimetro( (( base * 2 ) + ( altura * 2 )) );
}
@Override
public void calculaArea() {
setArea( (base * altura) );
}
@Override
public String toString() {
String dados;
dados = "\n-------------------------";
dados += "\nRetângulo:";
dados += "\n-------------------------";
dados += "\nAltura: "+ df.format(this.getAltura());
dados += "\nBase: "+ df.format(this.getBase());
dados += "\nÁrea: "+ df.format(this.getArea())+ " cm";
dados += "\nPerímetro: "+ df.format(this.getPerimetro())+ " cm";
return dados;
}
}
<file_sep>/Exercicios_Java_Orientacao_Objeto3/src/exercicios2/Loja.java
package exercicios2;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class Loja {
public static void main(String[] args) {
//Vector<Produto> produtos = new Vector<Produto>();
List<Produto> produtos = new ArrayList<>();
produtos.add(new CD("Nirvana - Bleach", 25.99d, 16, "01212689"));
produtos.add(new CD("Raimundos - Lavô Tá Novo", 15.99d, 12, "01288989"));
produtos.add(new CD("Raimundos - Lavô Tá Novo", 15.99d, 12, "01288989"));
produtos.add(new CD("Raimundos - Lavô Tá Novo", 15.99d, 12, "555877888"));
produtos.add(new DVD("Guia do Mochileiro das Galáxias", 59.9d, 180, "1478787"));
produtos.add(new DVD("Contatos de 4º Grau", 65.9d, 130, "8998914"));
produtos.add(new Livro("Como trabalhar para um idiota", "<NAME>", 36.85d, "14548777"));
produtos.add(new Livro("Guia do Mochileiro das Galáxias - Volume 1", "<NAME>", 24.75d, "59595984"));
produtos.add(new Livro("CADE", "<NAME>", 24.75d, "59595984"));
DVD dvd1 = new DVD("Guia do Mochileiro das Galáxias", 59.9d, 180, "1478787");
CD cd1 = new CD("Foo Fighters", 30.99, 15, "010106646");
// pesquisando um produto utilizando um objeto como parâmetro
//buscaProduto(produtos, dvd1);
// System.err.println(produtos.get(1).equals(produtos.get(3)));
// System.err.println(produtos.get(1).equals(produtos.get(2)));
ComparacaoImpl comparador = new ComparacaoImpl();
//comparador.buscaProdutoNomeCodBarras(produtos, "Cota");
for (Produto produto : produtos) {
System.out.println(produto.toString());
}
Collections.sort(produtos);
for (Produto produto : produtos) {
System.err.println(produto.toString());
}
}
public static void buscaProduto(List<Produto> vetorProdutos, Produto produtoPesquisado){
int tamanhoVetor = vetorProdutos.size();
int posicao = 0;
boolean encontrado = false;
for (Produto produtos : vetorProdutos) {
posicao++;
if ( produtos.equals(produtoPesquisado) ){
System.out.println("\nProduto encontrado. Posição: "+ (posicao-1));
System.out.println("---------------------------------------");
System.out.println(produtos.toString());
encontrado = true;
}else if ( (posicao == tamanhoVetor) && !encontrado) {
System.out.println("Produto não encontrado");
}
}
}
}
| 52a17da51f92931be9fe5e4bbf5cb06b16f25250 | [
"Java"
] | 6 | Java | william-marquetti/Exercicios_Java_Orientacao_Objeto3 | 5e05d02085a296a60e801dc32e2bebc73672425c | a3abec594b6f8518fe26314b8df7dde8ab75fed8 | |
refs/heads/master | <file_sep># TOPcalculator
The Odin Project Web Dev Calculator
<file_sep>$(document).ready(function(){
var subtotal = 0; // tracks the calculations on the background
var currOperator = "add"; // tracks the operations
var thread = [0]; // Displays on the main screen
var currThread = []; //Displays on the top screen
var comma = false; //tracks if a comma has been inserted
// When a number is pressed
$(".number").click(function(){
if (thread.length < 17){
thread.push(parseFloat($(this).val()));
if (thread.length==2 && thread[0]==0){
thread.shift();
}
};
$("#total").text(thread.join(""));
});
// When Plus, Minus, Multiply or Divide is pressed:
$(".operator").click(function(){
// Checks if the last key was an operator and updates it
if (parseFloat(thread.join("")) == 0) {
thread = [0];
currThread.pop();
currThread.push($(this).text());
currOperator = $(this).val();
$("#thread").text(currThread.join(""));
return 0;
}
// Performs the operation based on the last current Operator
currThread.push(parseFloat(thread.join(""))); // push value
currThread.push($(this).text()); // push operator
switch(currOperator){
case("add"):
subtotal += parseFloat(thread.join(""));
break;
case("subt"):
subtotal -= parseFloat(thread.join(""));
break;
case("mult"):
subtotal *= parseFloat(thread.join(""));
break;
case("divide"):
subtotal /= parseFloat(thread.join(""));
break;
}
comma = false;
currOperator = $(this).val();
thread = [0];
$("#total").text(subtotal);
$("#thread").text(currThread.join(""));
});
$("#dot").click(function(){
if (!comma) {
comma = true;
thread.push(".");
$("#total").text(thread.join(""));
} else {
document.getElementById('audio').play()
}
});
$("#CE").click(function(){
thread = [0];
comma = false;
$("#total").text("0");
});
$("#C").click(function(){
subtotal = 0;
thread = [0];
currOperator = "add";
currThread = [];
comma = false;
$("#total").text(0);
$("#thread").text("");
});
$("#posNeg").click(function(){
if (parseFloat(thread.join(""))== 0) return 0;
if (thread[0]=="-"){
thread.shift();
$("#total").text(thread.join(""));
} else {
thread.unshift("-");
$("#total").text(thread.join(""));
}
});
$("#backSpace").click(function(){
if (thread.length > 1){
thread.pop();
$("#total").text(thread.join(""));
} else {
thread = [0];
$("#total").text(thread.join(""));
}
});
$("#equal").click(function(){
switch(currOperator){
case("add"):
subtotal += parseFloat(thread.join(""));
break;
case("subt"):
subtotal -= parseFloat(thread.join(""));
break;
case("mult"):
subtotal *= parseFloat(thread.join(""));
break;
case("divide"):
subtotal /= parseFloat(thread.join(""));
break;
}
if (subtotal - Math.round(subtotal) == 0) { comma = false; }
currOperator = "add";
thread = [subtotal];
$("#total").text(subtotal);
subtotal = 0;
currThread = [];
$("#thread").text("");
});
});
| c74265184677c1d4f5e57bb0ea5f10dd15c1f5f4 | [
"Markdown",
"JavaScript"
] | 2 | Markdown | EdMagal/TOPcalculator | 6d5b799cb94dc47513cdfd2271f697c6b1541b90 | 587332127bd73843f75e6d44202e560a8a6fbb6c | |
refs/heads/master | <file_sep>from unittest import TestCase
import io
import json
from contextlib import redirect_stdout
import requests
import requests_mock
from ..fetch_facebook_posts_data import (fetch_node_feed, fetch_posts,
format_datetime_string,
clean_reactions_data)
from .constants import (
BASE_FB_URL, REACTIONS_URL, FACEBOOK_POSTS_ONE_RECORD_RAW_DATA,
FACEBOOK_POSTS_ONE_RECORD_CLEAN_DATA,
SAMPLE_COUNT, FACEBOOK_LAST_PAGE_RECORD_RAW_DATA,
FACEBOOK_LAST_PAGE_RECORD_CLEAN_DATA,
FACEBOOK_POSTS_MULT_RECORD_RAW_DATA,
FACEBOOK_POSTS_MULT_RECORD_CLEAN_DATA)
AUTH_TOKEN_INVALID_DATA = {
"error": {
"message": "Invalid OAuth access token.",
"type": "OAuthException",
"code": 190,
"fbtrace_id": "ADSG8pUqsRg"
}
}
def read_access_token():
with open('config.json') as config_file:
data = json.load(config_file)
return data['access_token']
def equal_dicts(d1, d2, ignore_keys=None):
if isinstance(ignore_keys, str):
ignore_keys = [ignore_keys, ]
ignored = set(ignore_keys)
for k1, v1 in d1.items():
if k1 not in ignored and (k1 not in d2 or d2[k1] != v1):
return False
for k2, _ in d2.items():
if k2 not in ignored and k2 not in d1:
return False
return True
def sysoutput_to_dicts(out):
return [json.loads(x) for x in out.getvalue().split('\n')[:-1]]
class TestFetchFacebookPostsData(TestCase):
def test_raise_error_for_non_200_response(self):
error_message = (
b'{"error":{"message":"Error validating access token:'
b' Session has expired on Saturday, 24-Mar-18 05:00:00 PDT. '
b'The current time is Saturday, 24-Mar-18 05:20:17 PDT.",'
b'"type":"OAuthException","code":190,"error_subcode":463,"'
b'fbtrace_id":"ACz1b5AhZtt"}}')
with requests_mock.Mocker() as m:
request_url = BASE_FB_URL + 'account_id' + REACTIONS_URL + 'AAA'
m.register_uri(
'GET',
request_url,
status_code=400,
content=error_message)
with self.assertRaises(requests.exceptions.HTTPError) as error_manager:
fetch_node_feed('account_id', access_token='AAA')
# It's given us a helpful exception
self.assertIn(
'400 Client Error: {"error":{"message":"Error validating access token',
error_manager.exception.__str__())
def test_dont_raise_error_for_200_response(self):
with requests_mock.Mocker() as m:
request_url = BASE_FB_URL + 'account_id' + REACTIONS_URL + 'AAA'
m.register_uri(
'GET', request_url, status_code=200, content=json.dumps(
FACEBOOK_POSTS_ONE_RECORD_RAW_DATA).encode('utf-8'))
response = fetch_node_feed('account_id', access_token='AAA')
self.assertEqual(FACEBOOK_POSTS_ONE_RECORD_RAW_DATA, response)
def test_can_write_schema(self):
out = io.StringIO()
with redirect_stdout(out):
with requests_mock.Mocker() as m:
request_url = BASE_FB_URL + 'account_id' + REACTIONS_URL + 'AAA'
m.register_uri(
'GET', request_url, status_code=200, content=json.dumps(
FACEBOOK_POSTS_ONE_RECORD_RAW_DATA).encode('utf-8'))
fetch_posts(node_id='account_id', access_token='AAA')
out_dicts = sysoutput_to_dicts(out)
row_types = [x['type'] for x in out_dicts]
# We have a schema record type
self.assertIn('SCHEMA', row_types)
def test_can_write_one_record(self):
out = io.StringIO()
with requests_mock.Mocker() as m:
request_url = BASE_FB_URL + 'officialstackoverflow' + REACTIONS_URL + 'AAA'
m.register_uri('GET', request_url, status_code=200, content=json.dumps(
FACEBOOK_POSTS_ONE_RECORD_RAW_DATA).encode('utf-8'))
with redirect_stdout(out):
fetch_posts('officialstackoverflow', 'AAA')
out_dicts = sysoutput_to_dicts(out)
row_types = [x['type'] for x in out_dicts]
# We wrote at least 1 record
self.assertIn('RECORD', row_types)
# And it contains our expected data
self.assertTrue(
equal_dicts(
out_dicts[1]['record'],
FACEBOOK_POSTS_ONE_RECORD_CLEAN_DATA['data'][0],
ignore_keys='created_time'))
def test_can_write_multiple_records(self):
out = io.StringIO()
with requests_mock.Mocker() as m:
request_url = BASE_FB_URL + 'officialstackoverflow' + REACTIONS_URL + 'AAA'
m.register_uri('GET', request_url, status_code=200, content=json.dumps(
FACEBOOK_POSTS_MULT_RECORD_RAW_DATA).encode('utf-8'))
with redirect_stdout(out):
fetch_posts('officialstackoverflow', 'AAA')
out_dicts = sysoutput_to_dicts(out)
row_types = [x['type'] for x in out_dicts]
# We wrote at least 1 record
self.assertIn('RECORD', row_types)
# And it contains both of our expected data points
self.assertTrue(
equal_dicts(
out_dicts[1]['record'],
FACEBOOK_POSTS_MULT_RECORD_CLEAN_DATA['data'][0],
ignore_keys='created_time'))
self.assertTrue(
equal_dicts(
out_dicts[2]['record'],
FACEBOOK_POSTS_MULT_RECORD_CLEAN_DATA['data'][1],
ignore_keys='created_time'))
def test_records_have_singer_format_string_times(self):
out = io.StringIO()
with requests_mock.Mocker() as m:
request_url = BASE_FB_URL + 'officialstackoverflow' + REACTIONS_URL + 'AAA'
m.register_uri('GET', request_url, status_code=200, content=json.dumps(
FACEBOOK_POSTS_ONE_RECORD_RAW_DATA).encode('utf-8'))
with redirect_stdout(out):
fetch_posts('officialstackoverflow', 'AAA')
out_dicts = sysoutput_to_dicts(out)
# It has been converted
self.assertNotEqual(
out_dicts[1]['record']['created_time'],
FACEBOOK_POSTS_ONE_RECORD_RAW_DATA['data'][0]['created_time'])
# to the format that Singer expects
self.assertEqual(out_dicts[1]['record']['created_time'],
'2018-03-19T18:17:00Z')
def test_format_datetime_string_correctly(self):
fb_format_datetime = '2018-03-19T18:17:00+0000'
formatted_datetime = format_datetime_string(fb_format_datetime)
self.assertEqual(formatted_datetime, '2018-03-19T18:17:00Z')
def test_invalid_config_access_token(self):
out = io.StringIO()
with requests_mock.Mocker() as m:
request_url = (BASE_FB_URL +
'officialstackoverflow/feed?access_token=AAA')
m.register_uri(
'GET',
request_url,
status_code=200,
content=json.dumps(AUTH_TOKEN_INVALID_DATA).encode('utf-8'))
with redirect_stdout(out):
with self.assertRaises(requests.exceptions.HTTPError) as error_manager:
fetch_posts('officialstackoverflow', 'AAA')
error_message = error_manager.exception.args[0]
self.assertIn('400 Client Error', error_message)
self.assertIn('Invalid OAuth access token', error_message)
class TestCanFetchPostStats(TestCase):
def test_can_get_reaction_count_from_original_api_call(self):
with requests_mock.Mocker() as m:
reactions_url = BASE_FB_URL + 'account_id' + REACTIONS_URL + 'AAA'
m.register_uri(
'GET', reactions_url, status_code=200, content=json.dumps(
FACEBOOK_POSTS_ONE_RECORD_RAW_DATA).encode('utf-8'))
response = fetch_node_feed('account_id', access_token='AAA')
reaction_labels = [
'none_reaction_count',
'sad_count',
'like_count',
'love_count',
'pride_count',
'total_reaction_count',
'haha_count',
'wow_count',
'thankful_count',
'angry_count']
for r in reaction_labels:
self.assertTrue(response['data'][0][r])
def test_can_get_clean_reaction_count(self):
reaction_labels = [
'none_reaction_count', 'sad_count', 'like_count', 'love_count',
'pride_count', 'total_reaction_count', 'haha_count', 'wow_count',
'thankful_count', 'angry_count'
]
reactions_data = {}
for reaction in reaction_labels:
reactions_data[reaction] = SAMPLE_COUNT
cleaned_data = clean_reactions_data(reactions_data)
# And our resulting data is flat
self.assertEqual(cleaned_data['none_reaction_count'], 566)
self.assertEqual(cleaned_data['sad_count'], 566)
def test_can_get_clean_comment_count(self):
reactions_data = {
"comment_count": {
"data": [
],
"summary": {
"order": "ranked",
"total_count": 7,
"can_comment": True
}
}
}
cleaned_data = clean_reactions_data(reactions_data)
# And our resulting data is flat
self.assertEqual(cleaned_data['comment_count'], 7)
class TestStateAndPagination(TestCase):
def test_can_write_state(self):
out = io.StringIO()
with redirect_stdout(out):
with requests_mock.Mocker() as m:
request_url = BASE_FB_URL + 'account_id' + REACTIONS_URL + 'AAA'
m.register_uri('GET', request_url, status_code=200, content=json.dumps(
FACEBOOK_POSTS_ONE_RECORD_RAW_DATA).encode('utf-8'))
fetch_posts(node_id='account_id', access_token='AAA')
out_dicts = sysoutput_to_dicts(out)
row_types = [x['type'] for x in out_dicts]
# We have a state record type
self.assertIn('STATE', row_types)
def test_can_start_pulling_data_at_a_certain_page(self):
out = io.StringIO()
with requests_mock.Mocker() as m:
# Our first request URL points at second_request_uri
self.assertEqual(
FACEBOOK_POSTS_ONE_RECORD_RAW_DATA['paging']['cursors']['after'],
'after_cursor_ZZZ')
first_request_url = (
BASE_FB_URL +
'account_id' +
REACTIONS_URL +
'AAA'
'&after=after_cursor_YYY')
m.register_uri(
'GET', first_request_url, status_code=200, content=json.dumps(
FACEBOOK_POSTS_ONE_RECORD_RAW_DATA).encode('utf-8'))
second_request_url = (
BASE_FB_URL +
'account_id' +
REACTIONS_URL +
'AAA'
'&after=after_cursor_ZZZ')
# Our second_request_uri does not have an after cursor marker
m.register_uri(
'GET', second_request_url, status_code=200, content=json.dumps(
FACEBOOK_LAST_PAGE_RECORD_RAW_DATA).encode('utf-8'))
with self.assertRaises(KeyError):
FACEBOOK_LAST_PAGE_RECORD_RAW_DATA['paging']['cursors']['after']
# Fetch posts, with a cursor that points at our first URL
with redirect_stdout(out):
fetch_posts(node_id='account_id', access_token='AAA',
after_state_marker='after_cursor_YYY')
out_dicts = sysoutput_to_dicts(out)
# It recorded the state as expected
row_messages = [x['record']['message']
for x in out_dicts if x['type'] == 'RECORD']
state_record = [x for x in out_dicts if x['type'] == 'STATE'][0]
# It fetched both of our messages
self.assertIn(
FACEBOOK_LAST_PAGE_RECORD_CLEAN_DATA['data'][0]['message'],
row_messages)
self.assertIn(
FACEBOOK_POSTS_ONE_RECORD_CLEAN_DATA['data'][0]['message'],
row_messages)
# And recorded the state of the first call
self.assertEqual(
state_record['value']['after'],
FACEBOOK_POSTS_ONE_RECORD_CLEAN_DATA['paging']['cursors']['after'])
<file_sep>from setuptools import setup, find_packages
setup(name="tap-facebook-posts",
version="0.0.5",
description="Singer.io tap for extracting posts data from the Facebook Graph API",
author="rowanv",
license='Apache 2.0',
url="https://github.com/rowanv",
classifiers=["Programming Language :: Python :: 3 :: Only"],
py_modules=["tap_facebook_posts"],
install_requires=[
'python-dateutil==2.6.0', # This is required by singer-python,
# without this being here explicitly, there are dependency issues
'requests',
'singer-python==5.0.12',
],
python_requires='>=3',
entry_points="""
[console_scripts]
tap-facebook-posts=tap_facebook_posts:main
""",
packages=["tap_facebook_posts"],
package_data = {
"schemas": ["tap_facebook_posts/schemas/*.json"]
},
include_package_data=True,
)
<file_sep>
BASE_FB_URL = 'https://graph.facebook.com/v2.11/'
REACTIONS_URL = (
'/posts?fields=created_time,story,message,'
'reactions.limit(0).summary(1).as(total_reaction_count),'
'reactions.type(NONE).limit(0).summary(1).as(none_reaction_count),'
'reactions.type(LIKE).limit(0).summary(1).as(like_count),'
'reactions.type(LOVE).limit(0).summary(1).as(love_count),'
'reactions.type(HAHA).limit(0).summary(1).as(haha_count),'
'reactions.type(WOW).limit(0).summary(1).as(wow_count),'
'reactions.type(SAD).limit(0).summary(1).as(sad_count),'
'reactions.type(ANGRY).limit(0).summary(1).as(angry_count),'
'reactions.type(THANKFUL).limit(0).summary(1).as(thankful_count),'
'reactions.type(PRIDE).limit(0).summary(1).as(pride_count),'
'comments.limit(0).summary(1).as(comment_count)'
'&limit=100&access_token='
)
# Create the test values that the API must return. We dynamically create
# the reactions variables for both a RAW_DATA variable that simulates the
# raw data returned by the API, and a CLEAN_DATA variable that simulates
# the flattened data that our module returns
SAMPLE_COUNT = {
"data": [
],
"summary": {
"total_count": 566,
"viewer_reaction": "NONE"
}
}
FACEBOOK_POSTS_ONE_RECORD_DATA = {
'data': [{
'created_time': '2018-03-19T18:17:00+0000',
'message': 'This is a test message',
'id': '11239244970_10150962953494971',
"comment_count": {
"data": [
],
"summary": {
"order": "ranked",
"total_count": 7,
"can_comment": True
}
},
}, ],
'paging': {
'cursors': {'before': 'before_cursor_AAA', 'after': 'after_cursor_ZZZ'},
'next': 'https://graph.facebook.com/v2.11/11239244970/feed?access_token=AAA'}
}
# Add in the reactions
REACTION_LABELS = [
'none_reaction_count', 'sad_count', 'like_count', 'love_count',
'pride_count', 'total_reaction_count', 'haha_count', 'wow_count',
'thankful_count', 'angry_count'
]
FACEBOOK_POSTS_ONE_RECORD_RAW_DATA = dict(FACEBOOK_POSTS_ONE_RECORD_DATA)
FACEBOOK_POSTS_ONE_RECORD_CLEAN_DATA = dict(FACEBOOK_POSTS_ONE_RECORD_DATA)
for reaction in REACTION_LABELS:
FACEBOOK_POSTS_ONE_RECORD_RAW_DATA['data'][0][reaction] = SAMPLE_COUNT
FACEBOOK_POSTS_ONE_RECORD_CLEAN_DATA['data'][0][reaction] = 400
FACEBOOK_POSTS_ONE_RECORD_CLEAN_DATA['data'][0]['comment_count'] = 7
FACEBOOK_LAST_PAGE_RECORD_DATA = {
'data': [{
'created_time': '2018-03-19T18:17:00+0000',
'message': 'This is a the last page record message',
'id': '11239244970_10150962953494971'}, ],
"comment_count": {
"data": [
],
"summary": {
"order": "ranked",
"total_count": 7,
"can_comment": True
}
},
'paging': {
'cursors': {'before': 'before_cursor_BBB'}, }
}
FACEBOOK_LAST_PAGE_RECORD_CLEAN_DATA = FACEBOOK_LAST_PAGE_RECORD_DATA.copy()
FACEBOOK_LAST_PAGE_RECORD_RAW_DATA = FACEBOOK_LAST_PAGE_RECORD_DATA.copy()
for reaction in REACTION_LABELS:
FACEBOOK_LAST_PAGE_RECORD_CLEAN_DATA['data'][0][reaction] = 400
FACEBOOK_LAST_PAGE_RECORD_RAW_DATA['data'][0][reaction] = SAMPLE_COUNT
FACEBOOK_LAST_PAGE_RECORD_CLEAN_DATA['data'][0]['comment_count'] = 7
FACEBOOK_POSTS_MULT_RECORD_DATA = {
'data': [{
'created_time': '2018-03-19T18:17:00+0000',
'message': 'This is a test message',
'id': '11239244970_10150962953494971',
}, {
'created_time': '2018-03-19T18:17:00+0000',
'message': 'This is a anothertest message',
'id': '11239244970_10150962953494111',
"comment_count": {
"data": [
],
"summary": {
"order": "ranked",
"total_count": 7,
"can_comment": True
}
},
}],
'paging': {
'cursors': {'before': 'before_cursor_AAA', 'after': 'after_cursor_ZZZ'},
'next': BASE_FB_URL + '11239244970' + REACTIONS_URL + 'AAA'}
}
FACEBOOK_POSTS_MULT_RECORD_RAW_DATA = FACEBOOK_POSTS_MULT_RECORD_DATA.copy()
FACEBOOK_POSTS_MULT_RECORD_CLEAN_DATA = FACEBOOK_POSTS_MULT_RECORD_DATA.copy()
for reaction in REACTION_LABELS:
FACEBOOK_POSTS_MULT_RECORD_RAW_DATA['data'][0][reaction] = SAMPLE_COUNT
FACEBOOK_POSTS_MULT_RECORD_RAW_DATA['data'][1][reaction] = SAMPLE_COUNT
FACEBOOK_POSTS_MULT_RECORD_CLEAN_DATA['data'][0][reaction] = 400
FACEBOOK_POSTS_MULT_RECORD_CLEAN_DATA['data'][1][reaction] = 400
FACEBOOK_POSTS_MULT_RECORD_CLEAN_DATA['data'][0]['comment_count'] = 7
FACEBOOK_POSTS_MULT_RECORD_CLEAN_DATA['data'][1]['comment_count'] = 7
<file_sep>autopep8==1.3.4
flake8==3.5.0
nose==1.3.7
singer-tools==0.4.1
requests-mock==1.4.0
<file_sep>#!/bin/bash
# Check that source has correct formatting
autopep8 tap_facebook_posts/*/*.py -r --diff
# More comprehensive static analysis, complexity check
flake8 tap_facebook_posts/<file_sep>import argparse
import json
import singer
from .fetch_facebook_posts_data import fetch_posts
logger = singer.get_logger()
def create_parser():
parser = argparse.ArgumentParser()
parser.add_argument(
'-c', '--config', help='Config file', required=True)
parser.add_argument(
'-s', '--state', help='State file')
return parser
def main():
parser = create_parser()
args = parser.parse_args()
with open(args.config) as config_file:
config = json.load(config_file)
required_config_vars = ['access_token', 'node_id', ]
for r in required_config_vars:
if r not in config:
logger.fatal("Missing required configuration keys: {}".format(r))
exit(1)
after_state_marker = None
if args.state:
with open(args.state) as state_file:
state = json.load(state_file)
after_state_marker = state['after']
try:
fetch_posts(config['node_id'], access_token=config['access_token'],
after_state_marker=after_state_marker)
except Exception as exception:
logger.critical(exception)
raise exception
if __name__ == "__main__":
main()
<file_sep>from unittest import TestCase
from .. import create_parser
class TestTapValid(TestCase):
def setUp(self):
self.parser = create_parser()
def test_can_call_tap_through_command_line(self):
parsed = self.parser.parse_args(['--config', 'config.json'])
self.assertTrue(parsed)
<file_sep>python-dateutil==2.6.0
requests==2.18.4
singer-python==5.0.15
<file_sep>import os
import json
import datetime
import requests
import singer
MAX_REQUEST_ITERATIONS = 50
BASE_FACEBOOK_GRAPH_API_URL = 'https://graph.facebook.com/v2.11/'
REACTIONS_URL = (
'/posts?fields=created_time,story,message,'
'reactions.limit(0).summary(1).as(total_reaction_count),'
'reactions.type(NONE).limit(0).summary(1).as(none_reaction_count),'
'reactions.type(LIKE).limit(0).summary(1).as(like_count),'
'reactions.type(LOVE).limit(0).summary(1).as(love_count),'
'reactions.type(HAHA).limit(0).summary(1).as(haha_count),'
'reactions.type(WOW).limit(0).summary(1).as(wow_count),'
'reactions.type(SAD).limit(0).summary(1).as(sad_count),'
'reactions.type(ANGRY).limit(0).summary(1).as(angry_count),'
'reactions.type(THANKFUL).limit(0).summary(1).as(thankful_count),'
'reactions.type(PRIDE).limit(0).summary(1).as(pride_count),'
'comments.limit(0).summary(1).as(comment_count)'
'&limit=100&access_token='
)
def get_abs_path(path):
return os.path.join(os.path.dirname(os.path.realpath(__file__)), path)
def load_schema(entity):
return singer.utils.load_json(get_abs_path(
"schemas/{}.json".format(entity)))
def format_datetime_string(original_dt):
"""Convert datetime into formatted string that is compatible w/ Singer spec.
"""
FB_DATETIME_FORMAT = "%Y-%m-%dT%H:%M:%S+%f"
TARGET_DATETIME_PARSE = "%Y-%m-%dT%H:%M:%SZ"
dt = datetime.datetime.strptime(original_dt, FB_DATETIME_FORMAT)
return dt.strftime(TARGET_DATETIME_PARSE)
def fetch_node_feed(node_id, access_token, *, after_state_marker=None):
"""Fetch the feed with all Post nodes for a given node.
For more info, see
https://developers.facebook.com/docs/graph-api/using-graph-api/
node_id: str or int, unique identifier for a given node.
access_token: str, access token to Facebook Graph API
after_state_marker: If provided, will only fetch posts after that marker.
"""
base_url = (BASE_FACEBOOK_GRAPH_API_URL + str(node_id) +
REACTIONS_URL + access_token)
if not after_state_marker:
url = base_url
else:
url = base_url + '&after=' + after_state_marker
response = requests.get(url)
if response.status_code == 200:
return json.loads(response.content.decode('utf-8'))
else:
error_message = (str(response.status_code) + ' Client Error: ' +
response.content.decode('utf-8'))
raise requests.exceptions.HTTPError(error_message)
def clean_reactions_data(record):
# Flatten the reactions data
reactions = [
'none_reaction_count', 'sad_count', 'like_count', 'love_count',
'pride_count', 'total_reaction_count', 'haha_count', 'wow_count',
'thankful_count', 'angry_count', 'comment_count',
]
for key, value in record.items():
if key in reactions:
try:
record[key] = record[key]['summary']['total_count']
except TypeError:
pass # record is already clean
return record
def write_records(data):
for record in data:
record['created_time'] = format_datetime_string(record['created_time'])
record = clean_reactions_data(record)
singer.write_record('facebook_posts', record)
def fetch_posts(node_id, access_token, after_state_marker=None):
"""Fetches all posts for a given node.
node_id: str or int, unique identifier for a given node.
access_token: str, access token to Facebook Graph API
after_state_marker: If provided, will only fetch posts after that marker.
"""
current_state_marker = after_state_marker
request_iterations_count = MAX_REQUEST_ITERATIONS
schema = load_schema("facebook_posts")
singer.write_schema("facebook_posts", schema, key_properties=["id"])
node_feed = fetch_node_feed(node_id, access_token, after_state_marker=after_state_marker)
write_records(node_feed['data'])
try:
while node_feed['paging']['cursors']['after'] and request_iterations_count:
response_after_state_marker = node_feed['paging']['cursors']['after']
# Did we already fetch the reponse marked by this state marker?
if current_state_marker != response_after_state_marker:
singer.write_state({'after': response_after_state_marker})
node_feed = fetch_node_feed(
node_id, access_token,
after_state_marker=response_after_state_marker)
write_records(node_feed['data'])
request_iterations_count -= 1
else:
break
except KeyError:
pass
<file_sep># tap-facebook-posts
Singer.io tap for getting Facebook posts from the Facebook Graph API.
[](https://badge.fury.io/py/tap-facebook-posts)
This tap:
- Pulls posts data for a given node from the [Facebook Graph API]. If no state is specified, it will pull all
available data for the feed, up to a limit of 50 API calls.
- Outputs the schema for the posts resource
- Prints the 'after' cursor (the next post that one should request from the API) to STATE
- Can fetch all posts after an 'after' cursor if provided with a `-s` flag
[Facebook Graph API]: https://developers.facebook.com/docs/graph-api
## Connecting tap-facebook-posts
### Quick Start
1. Install
We recommend using a virtualenv
```
virtualenv -p python3 my_venv
source my_venv/bin/activate
pip install tap-facebook-posts
```
2. Create the config file
Create a JSON file called `config.json`. Its contents should look like:
```
{
"node_id": "<NODE_ID>",
"access_token": "<YOUR_FACEBOOK_GRAPH_API_ACCESS_TOKEN>"
}
```
You can quickly get a Graph API Explorer access token via the [Graph API Explorer](https://developers.facebook.com/tools/explorer?method=GET&path=me%3Ffields%3Did%2Cname&version=v2.12)
3. Run the tap
```
tap-facebook-posts -c config.json
```
4. (Optional) If you'd like to run the tap with a STATE, you should make a JSON file called `state.json`. Its contents should look like:
```
{
"after": "<YOUR_FACEBOOK_AFTER_STATE_MARKER>"
}
```
You can then run the tap with
```
tap-facebook-posts -c config.json -s state.json
```
If you omit state settings, it will fetch all available posts for the given `node_id`.
## Development
### Running un-packaged tap
```
cd tap_facebook_posts
python . -c ../config.json
```
```
python -m tap_facebook_posts -c ../config.json
```
### Running tests
Install the requirements in `requirements-dev.txt`. Then, run the tests with `nosetests`.
You can check the tap with singer-tools using `singer-check-tap --tap tap-facebook-posts -c config.json`
### Linting
```
bash dev_tools/linter.sh
```
## Deploy
TestPyPI
```
python setup.py sdist
twine upload --repository-url https://test.pypi.org/legacy/ dist/*
pip install --index-url https://test.pypi.org/simple/ tap-facebook-posts==0.0.2a1
```
ProdPyPI
```
twine upload dist/*
``` | 25cb84e123336e092dbc373a8c71d3f77a27ce5a | [
"Markdown",
"Python",
"Text",
"Shell"
] | 10 | Python | rowanv/tap-facebook-posts | fa521e6f81be95e7a99feedef8d0fad94c52e71f | 726c1d2962101cb869badfe23f1e547b23b7c0bd | |
refs/heads/master | <file_sep># python sync tool
import os as os
import datetime
import time
import shutil
import pdb
class sync:
def __init__(self, source, destination ):
self.src = source
self.dest = destination
def checkRoot(self, src , dest):
if not os.path.exists(dest):
os.mkdir(dest)
def syncDir(self, src, dest):
srcList = os.listdir(src)
srcTime = [None]*len(srcList)
destList = os.listdir(src)
destTime = [None]*len(srcList)
for i in range(len(srcList)):
srcList[i] = src + srcList[i]
destList[i] = dest + destList[i]
srcTime[i] = os.path.getmtime(srcList[i])
print(srcList[i])
# IF THE DESTINATION PATH EXISTS
if os.path.exists(destList[i]) == True:
# pdb.set_trace()
if os.path.isfile(srcList[i]) == False:
os.chdir(srcList[i])
else:
pass
destTime[i] = os.path.getmtime(destList[i])
# IF THE DESTINATION IS OLDER
if destTime[i] < srcTime[i]:
try: # IF DESTINATION IS A DIRECTORY USE COPYTREE
shutil.rmtree(destList[i])
shutil.copytree(srcList[i], destList[i])
except OSError: # IF NOT, USE COPY2
shutil.copy2(srcList[i], destList[i])
else:
pass
else:
try:
shutil.copytree(srcList[i], destList[i])
except OSError:
shutil.copy2(srcList[i], destList[i])
return srcList, srcTime, destList, destTime
def main(self, src, dest):
self.checkRoot(src, dest)
srcList, srcTime, destList, destTime = self.syncDir(src,dest)
now = time.mktime(datetime.datetime.now().timetuple())
return srcList, srcTime, destList, destTime
#sy = sync('c:\users\Arl_guest\desktop','c:\users\Arl_guest\desktop\TEST')
# sy = sync('/home/michael/Dropbox/', '/home/michael/TEST/')
sy = sync('/users/michael/git/', '/users/michael/desktop/test/')
srcList, srcTime, destList, destTime = sy.main(sy.src, sy.dest)
print('Sync Finished')
<file_sep>
#program that backs up the specified directories to an external source
import shutil, errno, datetime, os, tarfile
def main():
#declare sources
C = '/C/Shares/'
#declare destination root
Save_root = r'/External_HDD/Backups/Server/'
#declare how long (in days) you want to keep daily backups
dailyDuration = 7
#declare what on which day you want to keep those past the above
keepDay = 'Sat' #Capitalize the first letter
#find out what day it is
today = str(datetime.date.today())
#make a new folder to house all the backups
save = Save_root+today+'_Backup'
os.mkdir(save)
Backup(C, save, 'Server')
# create tarball of the newly created folder
tar = tarfile.open(save+'.tar.bz2', 'w:bz2')
tar.add(save)
tar.close()
# delete the non-compressed file
os.rmtree(save)
## remove any old backups
clean_up(today, Save_root, dailyDuration, keepDay)
def Backup(source, destination_root, name):
save = destination_root +r'/'+ name
shutil.copytree(source, save)
def clean_up(today, directory, dailyDuration, keepDay):
os.chdir(directory)
age = datetime.datetime.now() - datetime.timedelta(days = dailyDuration)
for somefile in os.listdir('.'):
file_age = os.path.getmtime(somefile)
file_age = datetime.datetime.fromtimestamp(file_age)
file_day = file_age.strftime("%a")
#check if the file is older than specified date
#check if the file was created on the keep day
if file_age < age and file_day != keepDay:
if os.path.isfile(somefile) == True:
os.remove(somefile)
else:
os.rmdir(somefile)
else:
pass
main()
| cc2ce1227abb0abed5eba7072318735e13f84bcb | [
"Python"
] | 2 | Python | 1IfByLAN2IfByC/DirectorySync | 47c10bc03337c183b107dcab60945b664d8e342f | cd57c7b6241157a4d95a6b82d49ebbe899d5c74e | |
refs/heads/master | <repo_name>Techso/Tinder-Clone-App<file_sep>/src/App.js
import './App.css';
import Card from './components/Card';
import SwipeIcons from './components/SwipeIcons';
import Header from './components/Header';
function App() {
return (
<div className="app">
<Header/>
<Card/>
<SwipeIcons/>
</div>
);
}
export default App;
<file_sep>/src/components/SwipeIcons.js
import React from 'react'
import '../CustomStyles/SwipeIcons.css'
import {Replay, Close, StarRate, Favorite, FlashOn,} from "@material-ui/icons"
import { IconButton } from '@material-ui/core'
function SwipeIcons() {
return (
<div className="swipeIcons">
<IconButton className="swipeIconsRepeat">
<Replay fontSize="large"/>
</IconButton>
<IconButton className="swipeIconsLeft">
<Close fontSize="large"/>
</IconButton>
<IconButton className="swipeIconsStar">
<StarRate fontSize="large"/>
</IconButton>
<IconButton className="swipeIconsRight">
<Favorite fontSize="large"/>
</IconButton>
<IconButton className="swipeIconsLightening">
<FlashOn fontSize="large"/>
</IconButton>
</div>
)
}
export default SwipeIcons
| 2ac0efdc44e5efb1f7af98718079cdce3a3bbb4b | [
"JavaScript"
] | 2 | JavaScript | Techso/Tinder-Clone-App | 5bfd406eee287f812cf918270a0c7a59399c9f85 | 1d8799c074fb5ad9c0b7670d58357637fc6b5a56 | |
refs/heads/master | <repo_name>jbonigomes/popquiz<file_sep>/app/js/main.js
$('document').ready(function() {
// Let's make the page refreshes re-usable
function pickRandomQuestion() {
var data = questions[Math.floor(Math.random() * (questions.length))];
$('body').removeClass().addClass(data.color);
$('#question').removeClass().addClass(data.color);
$('#answers .container h2').html(data.answer);
$('#question .container h1').html(data.question);
$('h2').removeClass();
}
pickRandomQuestion();
// A couple of event listeners
$('#question .refresh').on('click', 'i', function() {
pickRandomQuestion();
});
$('#question').on('click', 'h1', function() {
$('h2').addClass('active');
});
// The all page
questions.forEach(function(question, i) {
$('#allquestions .container').append('<h2 class="active">' + (i+1) + ') ' + question.question + '</h2>');
$('#allquestions .container').append('<h3>' + question.answer + '</h3>');
});
});
| bf08a2737c8fcb9c79b0e32e8bcc0e549e0ea28e | [
"JavaScript"
] | 1 | JavaScript | jbonigomes/popquiz | f01a21eb800f7dda986ab49e0c5e38ccca7f67b5 | b69103d3fdb3da7acf461169fa2cffe2327ce61f | |
refs/heads/master | <repo_name>fcamposgonzlez/UnderMusic<file_sep>/okio-1.15.0/build.gradle
configurations.maybeCreate("default")
artifacts.add("default", file('okio-1.15.0.jar'))<file_sep>/app/src/main/java/com/example/undermusic/MainActivity.java
package com.example.undermusic;
import android.content.Intent;
import android.net.Uri;
import android.os.AsyncTask;
import android.os.Bundle;
import com.google.android.material.floatingactionbutton.FloatingActionButton;
import com.google.android.material.snackbar.Snackbar;
import android.view.View;
import com.google.android.material.navigation.NavigationView;
import androidx.core.view.GravityCompat;
import androidx.drawerlayout.widget.DrawerLayout;
import androidx.appcompat.app.ActionBarDrawerToggle;
import androidx.appcompat.app.AppCompatActivity;
import androidx.appcompat.widget.Toolbar;
import android.view.Menu;
import android.view.MenuItem;
import com.spotify.android.appremote.api.ConnectionParams;
import com.spotify.android.appremote.api.Connector;
import com.spotify.android.appremote.api.SpotifyAppRemote;
import com.spotify.protocol.client.Subscription;
import com.spotify.protocol.types.PlayerState;
import com.spotify.protocol.types.Track;
import com.spotify.sdk.android.authentication.AuthenticationClient;
import com.spotify.sdk.android.authentication.AuthenticationRequest;
import com.spotify.sdk.android.authentication.AuthenticationResponse;
import android.util.Log;
import android.widget.TextView;
import androidx.fragment.app.Fragment;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.Response;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.ExecutionException;
import org.json.JSONObject;
public class MainActivity extends AppCompatActivity
implements NavigationView.OnNavigationItemSelectedListener, GenerarPlaylist.OnFragmentInteractionListener,
Reproductor.OnFragmentInteractionListener,EditarGenero.OnFragmentInteractionListener,EditarArtista.OnFragmentInteractionListener {
private static final String CLIENT_ID = "43455e8ed37542e8b4a4ed8dc5df70ce";
private static final String REDIRECT_URI = "http://localhost:8888/callback";
public static SpotifyAppRemote mSpotifyAppRemote;
private static final int REQUEST_CODE = 1337;
private TextView txtShowTextResult;
private AuthenticationResponse response;
public static String playlistAct = "37i9dQZF1DX2sUQwD7tbmL";
public static List<String> ArtistasGeneral = new ArrayList<String>();
public static List<String> GeneroGeneral = new ArrayList<String>();
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent intent) {
super.onActivityResult(requestCode, resultCode, intent);
// Check if result comes from the correct activity
if (requestCode == REQUEST_CODE) {
this.response = AuthenticationClient.getResponse(resultCode, intent);
txtShowTextResult = findViewById(R.id.princi);
switch (response.getType()) {
// Response was successful and contains auth token
case TOKEN:
txtShowTextResult.setText("Conectado, Use el Menu para navegar.");
break;
// Auth flow returned an error
case ERROR:
// Handle error response
txtShowTextResult.setText("Error de Comunicación con Servidor");
break;
// Most likely auth flow was cancelled
default:
// Handle other cases
}
}
}
protected void onNewIntent(Intent intent) {
super.onNewIntent(intent);
Uri uri = intent.getData();
if (uri != null) {
AuthenticationResponse response = AuthenticationResponse.fromUri(uri);
switch (response.getType()) {
// Response was successful and contains auth token
case TOKEN:
// Handle successful response
break;
// Auth flow returned an error
case ERROR:
// Handle error response
break;
// Most likely auth flow was cancelled
default:
// Handle other cases
}
}
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
DrawerLayout drawer = (DrawerLayout) findViewById(R.id.drawer_layout);
ActionBarDrawerToggle toggle = new ActionBarDrawerToggle(
this, drawer, toolbar, R.string.navigation_drawer_open, R.string.navigation_drawer_close);
drawer.addDrawerListener(toggle);
toggle.syncState();
NavigationView navigationView = (NavigationView) findViewById(R.id.nav_view);
navigationView.setNavigationItemSelectedListener(this);
}
@Override
public void onBackPressed() {
DrawerLayout drawer = (DrawerLayout) findViewById(R.id.drawer_layout);
if (drawer.isDrawerOpen(GravityCompat.START)) {
drawer.closeDrawer(GravityCompat.START);
} else {
super.onBackPressed();
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
//noinspection SimplifiableIfStatement
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
@SuppressWarnings("StatementWithEmptyBody")
@Override
public boolean onNavigationItemSelected(MenuItem item) {
// Handle navigation view item clicks here.
int id = item.getItemId();
Fragment fragment = null;
Boolean fragmentoSelecionado = false;
int seleccionFrag = 0;
if (id == R.id.nav_camera) {
// Handle the camera action
fragment = new GenerarPlaylist();
((GenerarPlaylist) fragment).setResponse(this.response);
fragmentoSelecionado = true;
} else if (id == R.id.nav_gallery) {
fragment = new Reproductor();
fragmentoSelecionado = true;
} else if (id == R.id.nav_slideshow) {
fragment = new EditarArtista();
fragmentoSelecionado = true;
} else if (id == R.id.nav_manage) {
fragment = new EditarGenero();
fragmentoSelecionado = true;
} else if (id == R.id.nav_share) {
} else if (id == R.id.nav_send) {
}
if (fragmentoSelecionado){
getSupportFragmentManager().beginTransaction().replace(R.id.Contenedor, fragment).commit();
}
DrawerLayout drawer = (DrawerLayout) findViewById(R.id.drawer_layout);
drawer.closeDrawer(GravityCompat.START);
return true;
}
@Override
public void onFragmentInteraction(Uri uri) {
}
//SPOTIFY
@Override
protected void onStart() {
super.onStart();
// We will start writing our code here.
// Set the connection parameters
ConnectionParams connectionParams =
new ConnectionParams.Builder(CLIENT_ID)
.setRedirectUri(REDIRECT_URI)
.showAuthView(true)
.build();
SpotifyAppRemote.connect(this, connectionParams,
new Connector.ConnectionListener() {
@Override
public void onConnected(SpotifyAppRemote spotifyAppRemote) {
mSpotifyAppRemote = spotifyAppRemote;
mSpotifyAppRemote.getUserApi().getCapabilities().getRequestId();
Log.d("MainActivity", "Connected! Yay!");
// Now you can start interacting with App Remote
}
@Override
public void onFailure(Throwable throwable) {
Log.e("MainActivity", throwable.getMessage(), throwable);
// Something went wrong when attempting to connect! Handle errors here
}
});
AuthenticationRequest.Builder builder = new AuthenticationRequest.Builder(CLIENT_ID, AuthenticationResponse.Type.TOKEN, REDIRECT_URI);
builder.setScopes(new String[]{"user-read-recently-played","user-top-read","user-library-modify",
"user-library-read","playlist-read-private","playlist-modify-public","playlist-modify-private",
"playlist-read-collaborative","user-read-email","user-read-birthdate","user-read-private",
"user-read-playback-state","user-modify-playback-state","user-read-currently-playing",
"app-remote-control","streaming","user-follow-read","user-follow-modify"});
AuthenticationRequest request = builder.build();
AuthenticationClient.openLoginActivity(this, REQUEST_CODE, request);
}
private void connected() {
// Then we will write some more code here.
// Play a playlist
mSpotifyAppRemote.getPlayerApi().play("spotify:playlist:37i9dQZF1DX2sUQwD7tbmL");
}
@Override
protected void onStop() {
super.onStop();
// Aaand we will finish off here.
SpotifyAppRemote.disconnect(mSpotifyAppRemote);
}
}
| 0fa4bd949744fc840f1692f25761c169cfde7a88 | [
"Java",
"Gradle"
] | 2 | Gradle | fcamposgonzlez/UnderMusic | d35107179cf0ce2ac5bc5f5efc0d340d7df469e9 | 359201a34778dc3ee77c6214c0da48672dfdaa48 | |
refs/heads/main | <repo_name>arif-hstu/ema-john-server<file_sep>/app.js
/*process .env variables*/
require('dotenv').config();
const express = require('express');
const cors = require('cors');
const bodyParser = require('body-parser');
const app = express();
app.use(cors());
// parse application/x-www-form-urlencoded
app.use(bodyParser.urlencoded({ extended: false }))
app.use(bodyParser.json());
// import mongodb info
// require mongoClient
const MongoClient = require('mongodb').MongoClient;
const ObjectId = require('mongodb').ObjectID;
const password = '<PASSWORD>';
const uri = `mongodb+srv://arif-hstu:${process.env.DB_PASS}@<EMAIL>/{process.env.DB_NAME}?retryWrites=true&w=majority`;
const client = new MongoClient(uri, { useNewUrlParser: true, useUnifiedTopology: true });
// setting port
const port = 5000;
app.listen(process.env.PORT || port, () => {
console.log('liseting you')
});
/***********
*
* server requests go here
*
*************/
// deal with mongodb
client.connect(err => {
const productsCollection = client.db(process.env.DB_NAME).collection("products");
console.log('database connection successful');
// add a single product request
app.post('/addProduct', (req, res) => {
console.log(req.body)
productsCollection.insertOne(req.body);
res.send(JSON.stringify({message: 'Your product added to the Database!'}))
})
// get all products by request
app.get('/allProducts', (req,res) => {
productsCollection.find()
.toArray((err, documents) => {
res.send(documents)
})
})
})
app.get('/', (req, res) => {
res.send('Your api is working');
})
| 7f5713b4b538184519a92c5e33b97ddc9a0c8f25 | [
"JavaScript"
] | 1 | JavaScript | arif-hstu/ema-john-server | b4022c0adcacc5fed93976d338e93a4d3ac53f77 | 1aa264a62e30b51a52a4301be76fff97614c6fc4 | |
refs/heads/master | <file_sep>name = 'Notify'
icon = 'notification-new-symbolic'
def main(query):
if query.startswith('notify=') or query.startswith('n='):
infos = query[2:].split(':')
if len(infos) == 2:
cmd = "sleep " + infos[1] + '&& notify-send -i preferences-desktop-notification-bell "Custom Alert" "' + infos[0] + '"'
return 'Program notification: ' + infos[0], icon, cmd
else:
return 'Program notification: ' + query[2:], icon, 'echo "invialid command"'
else:
return 0
<file_sep>#!/usr/bin/python3
import gi
import os
import re
import sys
import threading
import subprocess
import setproctitle
gi.require_version('Gtk', '3.0')
from gi.repository import Gtk, Gdk, Pango, Gio
setproctitle.setproctitle('unam-launcher-service')
# Files
home = os.getenv("HOME") + '/'
applications = '/usr/share/applications/.'
running = home + '.config/unam/unam-launcher/running'
log_file = home + '.config/unam/unam-launcher/logs/log'
conf_file = home + '.config/unam/unam-launcher/config'
gconf = Gio.File.new_for_path(conf_file)
gfile = Gio.File.new_for_path(running)
gapps = Gio.File.new_for_path(applications)
monitor = gfile.monitor_file(Gio.FileMonitorFlags.NONE, None)
conf_changed = gconf.monitor_file(Gio.FileMonitorFlags.NONE, None)
dir_changed = gfile.monitor_directory(Gio.FileMonitorFlags.NONE, None)
#os.system("truncate -s 0 " + running)
def get_screen_size(x, y):
display = subprocess.Popen('xrandr | grep "\*" | cut -d" " -f4',shell=True,stdout=subprocess.PIPE).communicate()[0]
display = str(display.rstrip())[2:-1]
display = display.split('x')
if x == True and y == True:
return display
elif x == True and y == False:
return display[0]
elif x == False and y == True:
return display[1]
elif x == False and y == False:
return display[0] + 'x' + display[1]
def log(message):
with open(log_file, "a") as logfile:
logfile.write(message + '\n')
def str2bool(string):
return string.lower() in ("yes", "true", "t", "1")
class spacer():
def __init__(self):
self.box = Gtk.Box()
self.label = Gtk.Label('')
self.box.add(self.label)
self.label.set_hexpand(True)
self.label.set_alignment(0.1,0.5)
#self.box.set_sensitive(False)
def get_box(self):
return self.box
class extbutton():
def __init__(self):
self.box = Gtk.HBox()
self.label = Gtk.Label()
self.margin = Gtk.Label(' ')
self.icon = Gtk.Image()
self.label.set_hexpand(True)
self.icon.set_alignment(0,0.5)
self.label.set_alignment(0,0.5)
self.box.pack_start(self.margin, False, False, 4)
self.box.pack_start(self.icon, False, False, 4)
self.box.pack_start(self.label, True, True, 4)
def set_text(self, text):
self.label.set_text(text)
def set_font(self, name, size):
font_name = name + ' ' + str(size)
NewFont = Pango.FontDescription(font_name)
self.label.modify_font(NewFont)
def set_icon(self, icon_name, icon_size):
self.icon.set_from_icon_name(icon_name, icon_size)
def get_box(self):
return self.box
class appbutton():
def __init__(self):
self.button = Gtk.Button()
self.label = Gtk.Label()
self.icon = Gtk.Image()
self.layoutbox = Gtk.Box()
self.command = ''
self.build()
def build(self):
self.flat()
self.label.set_alignment(0,0.5)
self.button.set_hexpand(True)
self.layoutbox.pack_start(self.icon, False, False, 4)
self.layoutbox.pack_start(self.label, True, True, 4)
self.button.add(self.layoutbox)
def construct(self, icon_name, label_text, tooltip_text, command):
self.set_icon(icon_name, Gtk.IconSize.MENU)
self.set_label(label_text)
self.set_tooltip(tooltip_text)
self.set_command(command)
def flat(self):
classes = self.button.get_style_context()
classes.add_class('flat')
def on_click(self, button, cmd):
os.system(cmd + ' &')
log('App launched: ' + cmd)
app.invisible(None, None)
def set_icon(self, icon_name, icon_size):
self.icon.set_from_icon_name(icon_name, icon_size)
def get_icon(self):
return self.icon.get_from_icon_name()
def set_label(self, text):
self.label.set_text(text)
def get_label(self):
return self.label.get_text()
def set_tooltip(self, text):
self.button.set_tooltip_text(text)
def get_tooltip(self):
return self.button.get_tooltip_text()
def set_command(self, cmd):
self.command = cmd
self.button.connect('clicked', self.on_click, cmd)
def set_font(self, name, size):
font_name = name + ' ' + str(size)
NewFont = Pango.FontDescription(font_name)
self.label.modify_font(NewFont)
def get_command(self):
return self.command
def get_button(self):
return self.button
def get_basic_info(self):
return self.get_label(), self.get_tooltip()
def get_info(self):
return self.get_label(), self.get_command(), self.get_tooltip() #, self.get_icon()
# Main Program
class launcher(Gtk.Window):
def __init__(self):
Gtk.Window.__init__(self, title="Unam Launcher")
log('Started new service thread')
self.found = False
self.no_search = True
self.visible = False
self.config = conf_changed
self.trigger = monitor
self.update = dir_changed
# Extensions
self.cmdsearch = False
self.math = False
self.websearch = False
self.notify = False
self.runcmd = False
# keyboard shortcuts
accel = Gtk.AccelGroup()
accel.connect(Gdk.keyval_from_name('Q'), Gdk.ModifierType.CONTROL_MASK, 0, Gtk.main_quit)
self.add_accel_group(accel)
self.semaphore = threading.Semaphore(4)
self.wrapper = Gtk.VBox()
self.scrollbox = Gtk.ScrolledWindow()
self.scrollframe = Gtk.Viewport()
self.box = Gtk.Box(spacing=6)
self.spacer = Gtk.Box()
self.search_entry = Gtk.Entry()
self.app_menu = Gtk.VBox()
self.connect("delete-event", Gtk.main_quit)
self.connect('focus-out-event', self.invisible)
self.connect('key_press_event', self.on_key_press)
self.trigger.connect("changed", self.toggle_visible)
self.update.connect('changed', self.update_list)
self.config.connect('changed', self.load_config)
self.add(self.wrapper)
self.wrapper.pack_start(self.search_entry, False, False, 0)
# self.wrapper.pack_start(self.scrollbox, True, True, 0)
self.scrollbox.add(self.scrollframe)
self.scrollframe.add(self.box)
self.box.add(self.app_menu)
self.app_list = []
self.load_config(None, None, None, Gio.FileMonitorEvent.CHANGES_DONE_HINT)
self.con_search()
self.load_apps()
# self.assemble()
self.configure()
#self.clear()
def load_config(self, m, f, o, event):
if event == Gio.FileMonitorEvent.CHANGES_DONE_HINT:
buffer = open(conf_file, "r")
for line in buffer:
if line.startswith('CommandSearch'):
self.cmdsearch = str2bool(line.rstrip().split('=')[1])
if line.startswith('Math'):
self.math = str2bool(line.rstrip().split('=')[1])
elif line.startswith('WebSearch'):
self.websearch = str2bool(line.rstrip().split('=')[1])
elif line.startswith('Notify'):
self.notify = str2bool(line.rstrip().split('=')[1])
elif line.startswith('RunCmd'):
self.runcmd = str2bool(line.rstrip().split('=')[1])
def con_search(self):
#self.search_entry.set_placeholder_text('Enter program name')
self.search_entry.set_icon_from_icon_name(0, 'search')
self.search_entry.set_icon_tooltip_text(1, 'Search for Applications')
self.search_entry.connect('changed', self.search)
self.search_entry.connect('activate', self.launch)
BigFont = Pango.FontDescription("Sans Regular 14")
self.search_entry.modify_font(BigFont)
def update_list(self, m, f, o, event):
if event == Gio.FileMonitorEvent.CHANGES_DONE_HINT:
self.app_list = []
self.load_apps()
self.clear()
self.assemble()
def load_apps(self):
app_count = 0
for file in os.listdir(applications):
if os.path.isfile(os.path.join(applications, file)):
buffer = open(applications + '/' + file, "r")
name = "void"
icon = "void"
desc = "void"
cmd = "void"
for line in buffer:
if line.startswith("Icon="):
icon = line[5:].rstrip()
if line.startswith("Name="):
name = line[5:].rstrip()
if line.startswith("Comment="):
desc = line[8:].rstrip()
if line.startswith("Exec="):
cmd = line[5:].rstrip().lower()
cmd = cmd.replace("%u","")
cmd = cmd.replace("%f","")
if icon is not "void" and name is not "void" and cmd is not "void" and desc is not "void":
btn_app = appbutton()
btn_app.construct(icon, name, desc, cmd)
self.app_list.append(btn_app)
app_count += 1
icon = "void"
name = "void"
desc = "void"
cmd = "void"
def configure(self):
self.set_decorated(False)
self.resize(400,28)
#menu.set_size_request(350,5)
#menu.move(0,0)
self.set_skip_pager_hint(True)
self.set_skip_taskbar_hint(True)
self.set_position(Gtk.WindowPosition.CENTER_ALWAYS)
def clear(self):
for widget in self.app_menu:
self.app_menu.remove(widget)
def found_in(self, query, item):
if (self.cmdsearch):
if query in str(self.app_list[item].get_info()).lower():
return True
else:
if query in str(self.app_list[item].get_basic_info()).lower():
return True
return False
def populate(self):
apps = 0
query = self.search_entry.get_text()
if query != "":
for item in range(0, len(self.app_list)):
if self.found_in(query.lower(), item):
self.app_menu.pack_start(self.app_list[item].get_button(), True, True, 0)
apps += 1
self.found = True
if apps < 5 and apps > 0:
for item in range(0, 5 - apps):
space = spacer()
self.app_menu.pack_start(space.get_box(), True, True, 0)
elif apps == 0:
label = Gtk.Label('No apps found')
label.set_hexpand(True)
label.set_alignment(0.5,0)
if len(self.app_list) == 0:
self.found = False
self.app_menu.pack_end(label, True, True, 5)
def launch(self, *data):
if (self.found):
child = self.app_menu.get_children()
child[0].grab_focus()
self.activate_focus()
else:
self.invisible(None, None)
def search(self, entry):
if entry.get_text() != "":
if self.no_search == True:
self.no_search = False
self.wrapper.pack_start(self.scrollbox, True, True, 0)
self.found = False
self.clear()
self.resize(400, 170)
self.activate(entry.get_text())
self.populate()
self.show_all()
else:
self.wrapper.remove(self.scrollbox)
self.no_search = True
self.found = False
self.configure()
def assemble(self):
for item in range(0, len(self.app_list)):
self.app_menu.add(self.app_list[item].get_button())
def invisible(self, object, event):
self.hide()
self.visible = False
self.search_entry.set_text('')
self.no_search = True
self.wrapper.remove(self.scrollbox)
self.resize(400, 28)
def toggle_visible(self, m, f, o, event):
if event == Gio.FileMonitorEvent.CHANGES_DONE_HINT:
if self.visible:
self.invisible(None, None)
else:
self.visible = True
self.show_all()
self.set_focus()
def set_focus(self):
self.present()
self.set_modal(True)
self.set_keep_above(True)
def on_key_press(self, widget, event):
key = Gdk.keyval_name(event.keyval)
if 'Escape' in key:
print(str(key))
self.invisible(None, None)
def render_result(self, text, icon, cmd):
result = appbutton()
result.construct(text, text, icon, cmd)
result.set_label(str(text))
result.set_font("Sans Regular", 14)
result.set_icon(icon, Gtk.IconSize.MENU)
self.app_menu.add(result.get_button())
#### Extensions ####
def activate(self, input):
if (self.math):
import extensions.umath.math
math_module = extensions.umath.math
operation = math_module.main(input)
if operation != 0:
self.render_result(str(operation[0]), operation[1], operation[2])
self.found = True
return operation
if (self.websearch):
import extensions.websearch.search
web_module = extensions.websearch.search
operation = web_module.main(input)
if operation != 0:
self.render_result(operation[0], operation[1], operation[2])
self.found = True
if (self.notify):
import extensions.notify.notify
notif_module = extensions.notify.notify
operation = notif_module.main(input)
if operation != 0:
self.render_result(operation[0], operation[1], operation[2])
self.found = True
if (self.runcmd):
import extensions.run.runcmd
runcmd_module = extensions.run.runcmd
operation = runcmd_module.main(input)
if operation != 0:
self.render_result(operation[0], operation[1], operation[2])
self.found = True
app = launcher()
app.invisible(None, None)
Gtk.main()
<file_sep># unam-launcher
Simple and powerful launcher written in python
<file_sep>name = 'Math'
def main(query):
if query.startswith('='):
return (eval(query[1:]), 'gnome-calculator', 'gnome-calculator')
else:
return 0
<file_sep>name = 'RunCmd'
icon = 'gnome-terminal'
def main(query):
if query.startswith('cmd=') or query.startswith('c=') or query.startswith('command='):
command = query.split('=')
return 'Run command: ' + command[1], icon, '"' + command[1] + '"'
else:
return 0
<file_sep>name = 'WebSearch'
icon = 'web-browser'
def main(query):
if query.startswith('google=') or query.startswith('g='):
label = 'Search Google: '
infos = compose_url(query, 'https://google.com/search?q=')
return (label + infos[1], icon, 'firefox "' + infos[0] + '"')
elif query.startswith('wikipedia=') or query.startswith('w=') or query.startswith('wiki='):
label = 'Search Wikipedia: '
infos = compose_url(query, 'https://en.wikipedia.org/wiki/')
return (label + infos[1], icon, 'firefox "' + infos[0] + '"')
elif query.startswith('stackoverflow') or query.startswith('so='):
label = 'Search Stack Overflow: '
infos = compose_url(query, 'http://stackoverflow.com/search?q=')
return (label + infos[1], icon, 'firefox "' + infos[0] + '"')
elif query.startswith('duckduckgo=') or query.startswith('ddg='):
label = 'Search Duck Duck Go: '
infos = compose_url(query, 'https://duckduckgo.com/?q=')
return (label + infos[1], icon, 'firefox "' + infos[0] + '"')
else:
return 0
def compose_url(query, url):
search = query.split('=')[1]
url = url + search
return (url, search)
# if not os.path.exists(CACHE_DIR):
# os.makedirs(CACHE_DIR)
| 5727a2106ad15d1164c2a0cef1dbddd30b3692f4 | [
"Markdown",
"Python"
] | 6 | Python | Unam-OS/unam-launcher | 21dc43d2ce18daeed3192ded2253de8e5a2d301e | 56404663ed0f0f5d3126e338f85eebe818e46222 | |
refs/heads/master | <file_sep>using Business.Abstract;
using Business.Concrete;
using Business.DependencyResolvers.Ninject;
using DataAccess.Concrete.EntityFramework;
using Entities.Concrete;
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace WebFormsUI
{
public partial class ProductsForm : Form
{
public ProductsForm()
{
InitializeComponent();
//_productservice = new ProductManager(new EfProductDal());
_productservice = InstanceFactory.GetInstance<IProductService>();
//_categoryservice = new CategoryManager(new EfCategoryDal());
_categoryservice = InstanceFactory.GetInstance<ICategorService>();
}
private IProductService _productservice;
private ICategorService _categoryservice;
private void Form1_Load(object sender, EventArgs e)
{
LoadProducts();
LoadCategories();
}
private void LoadCategories()
{
cbxCategory.DataSource = _categoryservice.GetAll();
cbxCategory.DisplayMember = "CategoryName";
cbxCategory.ValueMember = "CategoryId";
cbxCategory2.DataSource = _categoryservice.GetAll();
cbxCategory2.DisplayMember = "CategoryName";
cbxCategory2.ValueMember = "CategoryId";
cbxCategoryUpdate.DataSource = _categoryservice.GetAll();
cbxCategoryUpdate.DisplayMember = "CategoryName";
cbxCategoryUpdate.ValueMember = "CategoryId";
}
private void LoadProducts()
{
dgwProduct.DataSource = _productservice.GetAll();
}
private void cbxCategory_SelectedIndexChanged(object sender, EventArgs e)
{
try
{
dgwProduct.DataSource = _productservice.GetProductsByCategory(Convert.ToInt32(cbxCategory.SelectedValue));
}
catch (Exception)
{
}
}
private void tbxProductName_TextChanged(object sender, EventArgs e)
{
if (!String.IsNullOrEmpty(tbxProductName.Text))
{
dgwProduct.DataSource = _productservice.GetProductsByProductName(tbxProductName.Text);
}
else
{
LoadProducts();
}
}
private void btnAdd_Click(object sender, EventArgs e)
{
try
{
_productservice.Add(new Product
{
CategoryId = Convert.ToInt32(cbxCategory2.SelectedValue),
ProductName = tbxUrunAdi2.Text,
QuantityPerUnit = tbxQuantity.Text,
UnitPrice = Convert.ToDecimal(tbxUnitPrice.Text),
UnitsInStock = Convert.ToInt16(tbxStock.Text)
});
MessageBox.Show("Yeni Ürün Eklendi");
LoadProducts();
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
private void btnUrunGuncelle_Click(object sender, EventArgs e)
{
try
{
_productservice.Update(new Product
{
ProductId = Convert.ToInt32(dgwProduct.CurrentRow.Cells[0].Value),
ProductName = tbxProductNameUpdate.Text,
CategoryId = Convert.ToInt32(cbxCategory2.SelectedValue),
UnitsInStock = Convert.ToInt16(TbxStockUpdate.Text),
QuantityPerUnit = TbxQuantityUpdate.Text,
UnitPrice = Convert.ToDecimal(TbxUnitPriceUpdate.Text)
});
MessageBox.Show("Ürün Güncellendi");
LoadProducts();
}
catch (Exception)
{
MessageBox.Show("Hata! Ürün güncellenemedi");
}
}
private void dgwProduct_CellClick(object sender, DataGridViewCellEventArgs e)
{
//seçilen ürün update için kutucukların dolması gerekir.
tbxProductNameUpdate.Text = dgwProduct.CurrentRow.Cells[1].Value.ToString();
cbxCategoryUpdate.SelectedValue = dgwProduct.CurrentRow.Cells[2].Value;
TbxUnitPriceUpdate.Text = dgwProduct.CurrentRow.Cells[3].Value.ToString();
TbxQuantityUpdate.Text = dgwProduct.CurrentRow.Cells[4].Value.ToString();
TbxStockUpdate.Text = dgwProduct.CurrentRow.Cells[5].Value.ToString();
}
private void btnRemove_Click(object sender, EventArgs e)
{
try
{
if (dgwProduct.CurrentRow != null)
{
_productservice.Delete(new Product
{
ProductId = Convert.ToInt32(dgwProduct.CurrentRow
.Cells[0].Value)
});
MessageBox.Show("Ürün Silme işlemi başarılı");
LoadProducts();
}
}
catch (Exception)
{
MessageBox.Show("Ürün Silinemedi");
}
}
}
}
<file_sep>using DataAccess.Abstract;
using Entities.Concrete;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Linq.Expressions;
using System.Text;
using System.Threading.Tasks;
namespace DataAccess.Concrete.EntityFramework
{
public class EfProductDal:EfEntityRepositoryBase<Product,ProjeContext> ,IProductDal
{
}
}
| 0db2b3ee97115190ca1b9850886b6a9bf25b0775 | [
"C#"
] | 2 | C# | aliclk/NLayeredApp | 3d5a5667d3f8577772ec06cae9c86aa5d4124079 | b037fbcd6e98e8eaa0076378e2fea83ee6fc9a35 | |
refs/heads/master | <file_sep>const path = require('path');
const MiniCssExtractPlugin = require('mini-css-extract-plugin');
module.exports = dirName => ({
entry: {
app: ['./src/app.tsx'],
vendor: Object.keys(require(`${dirName}/package`).dependencies),
},
output: {
filename: 'app.[hash].js',
publicPath: '/',
},
devServer: {
historyApiFallback: true,
port: 3300,
},
devtool: "source-map",
node: {
fs: 'empty',
net: 'empty',
module: 'empty',
},
resolve: {
extensions: [".ts", ".tsx", ".js", ".json"],
},
module: {
noParse: content => /express/.test(content),
rules: [
{
test: /\.tsx?$/,
use: ['awesome-typescript-loader'],
include: [
path.join(dirName, 'src'),
],
exclude: [
path.join(dirName, 'node_modules'),
],
},
{ enforce: "pre", test: /\.jsx?$/, loader: 'source-map-loader' },
{
test: /\.scss$/,
exclude: [
path.join(dirName, 'node_modules'),
],
use: [
'style-loader',
MiniCssExtractPlugin.loader,
'css-loader',
'sass-loader',
],
},
{
test: /\.css$/,
use: [
'style-loader',
MiniCssExtractPlugin.loader,
'css-loader',
],
},
{
test: /\.jp(e)?g|\.png/,
loader: 'file-loader',
options: {
name: '[name]-[hash].[ext]',
},
},
{
test: /\.woff2?$|\.ttf$|\.eot$|\.svg$/,
loader: 'url-loader',
options: {
name: '[name]-[hash].[ext]',
limit: 100000,
},
},
],
},
optimization: {
runtimeChunk: false,
splitChunks: {
cacheGroups: {
vendor: {
chunks: 'all',
name: 'vendor',
test: /node_modules\/(.*)\.js/,
},
},
},
},
}); | 3ad9459020bbe8d1fc70012199e0ef967eacba14 | [
"JavaScript"
] | 1 | JavaScript | YegorLuz/ReactShop | 4133c33d39b989883ea4e2e8f9aa72d520835ffd | 6da8d8bb9d5c769621da36df66275094e97e81b7 | |
refs/heads/main | <repo_name>Remkudusov/django-contract-search-website<file_sep>/README.md
# django-contract-search-website
This is my website for searching data about contracts in test workflow. Here is work with Django framework, REST API and more.
| 8137ab63b4d1d6919e8fe0416a3332761d6cf9c5 | [
"Markdown"
] | 1 | Markdown | Remkudusov/django-contract-search-website | 1c22b728c7306c1a07bcfd0ed3cf1474c5111887 | de8eb4553164681492dd14f7a0482f6609a6b1ea | |
refs/heads/master | <file_sep># Color's Editor :art:
Aplicação desenvolvida para o trabalho prático do Módulo 01 do Bootcamp Desenvolvimento Fullstack disponibilizado pelo Instituto de Gestão e Tecnologia da Informação - IGTI. Visando desenvolver as habilidades em JavaScript, HTML e CSS e manipulação do DOM, desenvolveu-se uma aplicação que realiza a alteração de cores em elementos através da seleção dos valores via input's do tipo range.
As cores seguem o formato RGB.
<hr>
<p align="center">
<img width="900px" src="https://github.com/ChristopherHauschild/colors-editor-bootcamp-fullstack/blob/master/CE.gif?raw=true"/>
</p>
## Linguagens e Tecnologias utilizadas: :computer:
<ul>
<li> HTML </li>
<li> CSS </li>
<li> JavaScript </li>
</ul>
## Instalação: :rocket:
Como se trata de uma página Web local, basta abrir o arquivo ```index.html``` no browser de preferência.
Aconselha-se a utilização do live-server, um servidor com auto refresh.
Para instalar basta seguir o comando ```npm install live-server```. E então rodar ```live-server``` na pasta raíz.
Esse procedimento pode ser realizado ainda através da extensão Live Server no VSCode.
### Maiores informações: :pencil:
As demais atividades e projetos desenvolvidos durante o Bootcamp podem ser encontradas neste <a href="https://github.com/ChristopherHauschild/bootcamp-fullstack-igti">link</a>
<hr>
Desenvolvido em frente ao :computer: por <NAME>
<file_sep>window.addEventListener('load', start)
let count = 0
let inputRed = null
let inputGreen = null
let inputBlue = null
function start() {
inputRed = document.querySelector('#inputRed')
inputRed.addEventListener('change', colorRedDiv)
inputGreen = document.querySelector('#inputGreen')
inputGreen.addEventListener('change', colorGreenDiv)
inputBlue = document.querySelector('#inputBlue')
inputBlue.addEventListener('change', colorBlueDiv)
buttonDark = document.querySelector('.dark')
buttonDark.addEventListener('click', enabledDark)
}
function colorRedDiv(event) {
let valueRed = document.querySelector('#valueRed')
valueRed.classList.add("inputActive")
valueRed.value = event.target.value
valueRed.addEventListener('change', setColors())
}
function colorGreenDiv(event) {
let valueGreen = document.querySelector('#valueGreen')
valueGreen.classList.add("inputActive")
valueGreen.value = event.target.value
valueGreen.addEventListener('change', setColors())
}
function colorBlueDiv(event) {
let valueBlue = document.querySelector('#valueBlue')
valueBlue.classList.add("inputActive")
valueBlue.value = event.target.value
valueBlue.addEventListener('change', setColors())
}
function setColors(R, G, B) {
let R = valueRed.value
let G = valueGreen.value
let B = valueBlue.value
let square = document.querySelector('.square')
square.style.backgroundColor = `rgb(${R}, ${G}, ${B})`
let h1 = document.querySelector('h1')
h1.style.color = `rgb(${R}, ${G}, ${B})`
let container = document.querySelector('.container')
container.style.boxShadow = `1px 2px 2px 0px rgb(${R}, ${G}, ${B})`
}
function enabledDark(event) {
count++
body = document.querySelector('body')
if (count % 2 !== 0) {
body.style.background = 'radial-gradient(circle, rgba(88,85,85,1) 0%, rgba(32,35,42,1) 100%)'
buttonDark.textContent = 'Light'
buttonDark.style.border = '2px solid yellow'
let container = document.querySelector('.container')
container.style.boxShadow = '3px 3px 3px 0px #fff'
} else {
console.log('ei')
body.style.background = '#0002'
buttonDark.textContent = 'Dark'
buttonDark.style.border = '1px solid #0001'
}
}
| 4bc806547908d7deb5ec6687fb28d4b8cf2a2f4d | [
"Markdown",
"JavaScript"
] | 2 | Markdown | ChristopherHauschild/colors-editor-bootcamp-fullstack | 1bf9df71972dcf68237ce4b8944d8d04e574f54f | 230e52a35931eee11af445f881718b57ca750c60 | |
refs/heads/master | <repo_name>PrasadSalimath/Angular-AlbumStoreProductPage<file_sep>/src/app/app.module.ts
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { FormsModule } from '@angular/forms';
import { HttpModule } from '@angular/http';
import { RouterModule, Routes } from '@angular/router'
import { AppComponent } from './app.component';
import { ProductPageComponent } from './product-page/product-page.component';
import { ProductDescriptionComponent } from './product-description/product-description.component';
import { ProductService } from './product.service';
import { ProductTracklistingComponent } from './product-tracklisting/product-tracklisting.component';
import { ProductListComponent } from './product-list/product-list.component';
import { AuthenticatedUserComponent } from './authenticated-user/authenticated-user.component';
import { SigninComponent } from './signin/signin.component'
const appRoutes: Routes = [
{ path: 'signin', component: SigninComponent },
{ path: 'authenticated', component: AuthenticatedUserComponent,
children:[
{ path: 'products', component: ProductListComponent},
{ path: 'product/:id', component: ProductPageComponent},
] },
{ path: '', component: SigninComponent},
{ path: '**', component: SigninComponent }
// { path: '', redirectTo: 'signin', pathMatch: 'full'}
]
@NgModule({
declarations: [
AppComponent,
ProductPageComponent,
ProductDescriptionComponent,
ProductTracklistingComponent,
ProductListComponent,
AuthenticatedUserComponent,
SigninComponent
],
imports: [
BrowserModule,
FormsModule,
HttpModule,
RouterModule.forRoot(appRoutes)
],
providers: [ProductService],
bootstrap: [AppComponent]
})
export class AppModule { }
<file_sep>/src/app/product.ts
export interface Product {
id: number;
artistName: string;
albumName: string;
}
| 61933538e08fd83a0b8505027b6ca62140f43588 | [
"TypeScript"
] | 2 | TypeScript | PrasadSalimath/Angular-AlbumStoreProductPage | d028ac5c140f14c24cda5ecf462f4e051f847ee6 | 9fb0cc826abf79e8939ca1f5fae495fc8c2fc8ee | |
refs/heads/master | <repo_name>dragosboca/dotmanager<file_sep>/lib/dotmanager/repo.rb
require 'dotmanager/config'
require 'dotmanager/util'
require 'git'
module Dotmanager
class Repo
extend self
def add_file(filename)
config = Config.instance
repobase = config[:base_repo]
g = Git.open repobase, :log => (Logger.new STDOUT)
g.add filename # add the file
config.files.each do |dbfile|
g.add dbfile
end # also add the database in the same commit
g.commit "add #{filename} to the database"
end
def remove_file(filename)
config = Config.instance
repobase = config[:base_repo]
g = Git.open repobase, :log => (Logger.new STDOUT)
g.remove filename # add the file
config.files.each do |dbfile|
g.add dbfile
end # also add the database in the same commit
g.commit "remove #{filename} from the database"
end
end
end
<file_sep>/lib/dotmanager.rb
require 'dotmanager/version'
require 'dotmanager/commands'
require 'dotmanager/config'
require 'dotmanager/repo'
require 'dotmanager/util'
module Dotmanager
# Your code goes here...
end
<file_sep>/lib/dotmanager/util.rb
require 'fileutils'
module Dotmanager
module Util
extend self
def abspath(source)
File.expand_path source
end
end
end
<file_sep>/Gemfile
source 'https://rubygems.org'
# Specify your gem's dependencies in dotmanager.gemspec
gemspec
<file_sep>/lib/dotmanager/commands.rb
require 'git'
require 'fileutils' # mv, ln, cp,...
require 'dotmanager/config'
require 'dotmanager/util'
require 'dotmanager/repo'
module Dotmanager
class Action
# create a new symlink, add the symlink to the database and add the new file to git repo
def create_symlink(source, destination, **options)
config = Config.instance
fail "Unexistent source file: #{source}" unless (FileUtils.stat source).file?
fail "File: #{source} or #{destination} already in database" if Config.source_defined?(source) ||
Config.destination_defined?(destination)
begin
FileUtils.ln_s Util.abspath(source), Util.abspath(destination)
rescue Errno::EEXIST
raise 'Destination file already in the repo. Maybe you should use install or force'
end
# now if the symlink suceeded
config.add_symlink source, destination, options[:description] rescue 'No description'
Repo.add_file destination
config.save_database
end
def delete_existing_symlink(source, **options)
config = Config.instance
fail "The file: #{source} is not in the database" unless Config.source_defined? Util.abspath(source)
fail "The file: #{source} is registered in the database but is not a symlink." unless File.stat(source).symlink?
destination = config.get_destination source
FileUtils.rm Util.abspath source
FileUtils.mv Util.abspath(destination) , Util.abspath(source)
if options[:remove_from_git] # also update the db
Repo.remove_file Config.get_destination source
config.remove_source source # we must do
end
config.remove_source source if options[:update_db]
end
def install_existing_symlink(source, **_options)
config = Config.instance
fail "Undefined source: #{source}" unless config.source_defined? source
destination = Config.get_destination source
if FileUtils.stat(Util.abspath source).file?
puts "#{source} already exists. Creating backup" #TODO replace with logger
backup_file source
end
FileUtils.symlink Util.abspath(source), Util.abspath(destination)
end
def check_symlink(source)
config = Dotmanager::Config.instance
return unless config.source_defined? source
def_source = Util.abspath config.get_destination source
def_destination = Util.abspath config.get_destination def_source
File.readlink def_source == def_destination
end
# block will be called for every bad defined link
def check_all_symlinks
config = Config.instance
config[:symlinks].each do |link|
unless check_symlink link[:src]
if block_given?
yield link
else
fail "Incorrect link #{link[:src]}"
end
end
end
end
def install_all_symlinks # This is part of bootstrap process (if ever run)
check_all_symlinks do |link| # check for the missing symlinks
install_existing_symlink link[:src] # if there is one than install it
end
end
def clone_repo(repo)
config = Config.instance
fail "Undefined repo #{repo}" unless config.repo_defined? repo
Git.clone(repo[:repo], repo[:name], :path=>repo[:dest], :branch=>(repo[:branch] rescue 'master')) # just clone the repo
end
def install_package(_package)
end
def uninstall_package(_package)
end
def install_all_packages
end
def install_fonts
end
private
def download_file(_url, _dest)
end
def backup_file(filename)
FileUtils.mv Util.abspath(filename), Util.abspath("#{filename}.dotbackup")
end
end
class Process
def bootstrap
end
def new_env
end
end
end
<file_sep>/lib/dotmanager/config.rb
require 'singleton'
require 'yaml'
require 'dotmanager/util'
# from https://gist.github.com/morhekil/998709/aafd65c5780a2ce3cda3ef020b6e5dead7cef1d0
# Symbolizes all of hash's keys and subkeys.
# Also allows for custom pre-processing of keys (e.g. downcasing, etc)
# if the block is given:
#
# somehash.deep_symbolize { |key| key.downcase }
#
# Usage: either include it into global Hash class to make it available to
# to all hashes, or extend only your own hash objects with this
# module.
# E.g.:
# 1) class Hash; include DeepSymbolizable; end
# 2) myhash.extend DeepSymbolizable
module DeepSymbolizable
def deep_symbolize(&block)
method = self.class.to_s.downcase.to_sym
syms = DeepSymbolizable::Symbolizers
syms.respond_to?(method) ? syms.send(method, self, &block) : self
end
module Symbolizers
extend self
# the primary method - symbolizes keys of the given hash,
# preprocessing them with a block if one was given, and recursively
# going into all nested enumerables
def hash(hash, &block)
hash.inject({}) do |result, (key, value)|
# Recursively deep-symbolize subhashes
value = _recurse_(value, &block)
# Pre-process the key with a block if it was given
key = yield key if block_given?
# Symbolize the key string if it responds to to_sym
sym_key = key.to_sym rescue key
# write it back into the result and return the updated hash
result[sym_key] = value
result
end
end
# walking over arrays and symbolizing all nested elements
def array(ary, &block)
ary.map { |v| _recurse_(v, &block) }
end
# handling recursion - any Enumerable elements (except String)
# is being extended with the module, and then symbolized
def _recurse_(value, &block)
if value.is_a?(Enumerable) && !value.is_a?(String)
# support for a use case without extended core Hash
value.extend DeepSymbolizable unless value.class.include?(DeepSymbolizable)
value = value.deep_symbolize(&block)
end
value
end
end
end
module Dotmanager
class Config
attr_reader :files
include Singleton
def initialize
@files = []
@config = {}
@config.extend DeepSymbolizable
end
# noinspection RubyScope
def load_file(filename)
file = Util.abspath(filename)
unless @files.include? file
@files << file
data = YAML.load_file(file) # transform the keys in symbols
merger = proc { |_, v1, v2| Hash === v1 && Hash === v2 ? v1.merge(v2, &merger) : v2 }
@config.merge! data, &merger
@config = @config.deep_symbolize
end if File.exist?(file)
@files.include? file
end
def [](key)
@config[key]
end
def source_defined?(source)
!@config[:symlinks].find { |s| s[:source] == source }.nil?
end
def destination_defined?(destination)
!@config[:symlinks].find { |s| s[:destination] == destination }.nil?
end
def get_destination(source)
fail "Undefined source: #{source}" unless source_defined? source
(@config[:symlinks].find { |s| s[:source] == source })[:destination].strip
end
def get_source(destination)
fail "Undefined destination: #{destination}" unless destination_defined? destination
(@config[:symlinks].find { |s| s[:destination] == destination })[:source].strip
end
def add_symlink(source, destination, description)
@config[:symlinks] << { description: description, source: source, destination: destination }
end
def delete_source_symlink(source)
fail "Undefined source: #{source}" unless source_defined? source
@config[:symlinks].reject! { |s| s[:source] == source }
end
def delete_destination_symlink(destination)
fail "Undefined destination: #{destination}" unless destination_defined? destination
@config[:symlinks].reject! { |s| s[:destination] == destination }
end
def repo_defined?(repo)
!config[:git_repos].find { |r| r[:repo] == repo }.nil?
end
def get_repo_dest(repo)
fail "Undefined repo #{repo}" unless repo_defined? repo
(@config[:git_repos].find {|r| r[:repo] == repo})[:dest]
end
def save_database
end
end
end
| 3aad00284370b9b117425ba2b4f846f413dbc0d4 | [
"Ruby"
] | 6 | Ruby | dragosboca/dotmanager | bf38dc5b57169dcbb755e058d1ff19644d0b157a | e0131366954b137c5091781308ead1a66051e195 | |
refs/heads/master | <file_sep>import app from './app';
/**
* List available serial ports
*/
app
.command('ports')
.description('list available serial ports')
.action(() => {
app.listSerialPorts();
});
/**
* Get chip id
*/
app
.command('cid')
.description('get device chip id')
.option('-p, --port <port>', 'device port')
.action((options) => {
app.getChipId(options.port);
});
/**
* Firmware upload
*/
app
.command('upload')
.description('compile and upload firmware')
.option('-p, --port <port>', 'device port')
.option('-d, --dir <dir>', 'firmware directory')
.option('-l, --lock <chip_id>', 'lock firmware to specific chip id')
.option('-s, --slaves <slaves>', 'maximum number of slaves allowed')
.option('-w --webui', 'upload web-ui after uploading the firmware image')
.option('-t --trial <time>', 'enable trial mode')
.option('-a --wizard', 'run firmware upload wizard')
.action((options) => {
app.uploadFirmware(options);
});
/**
* Flash erase
*/
app
.command('erase')
.description('erase device flash')
.option('-p, --port <port>', 'device port')
.action((options) => {
app.eraseFlash(options.port);
});
app
.command('config')
.description('configuration')
.action(() => {
app.showConfigMenu();
});
// Start application. Always run this at the end of this file.
app.start();
<file_sep>import chalk from 'chalk';
import clear from 'clear';
import Table from 'cli-table';
import { Command } from 'commander';
import Configstore from 'configstore';
import figlet from 'figlet';
import inquirer from 'inquirer';
import _ from 'lodash';
import helper, { BuildOptions, GitHubAuth } from 'mbox-builder-helper';
import ora from 'ora';
import readPkgUp from 'read-pkg-up';
import { Port } from './model';
import * as prompts from './prompt';
class App extends Command {
private static instance: App;
private pkg: readPkgUp.NormalizedReadResult;
private config: Configstore;
/**
* Class constructor
* @param appName
*/
private constructor() {
// read data from package.json
const _pkgUp = readPkgUp.sync({
cwd: __filename,
});
// get app's bin name from package.json
const appBinName = _.keys(_pkgUp.packageJson.bin)[0];
// call super
super(appBinName);
// copy local _pkg to class scoped pkg object
this.pkg = _pkgUp;
// create config store
this.config = new Configstore(this.pkg.packageJson.name);
// clear console
clear();
// show top caption
console.log(
chalk.cyanBright(
figlet.textSync('MBox Builder', { horizontalLayout: 'full' })
)
);
}
/**
* Store github credentials
* @param username
* @param password
*/
private storeGithubLogin(username: string, password: string): boolean {
this.config.set('gh_username', username);
this.config.set('gh_password', password);
return true;
}
/**
* Retrieve stored github credentials
* @param username
* @param password
*/
private getGithubLogin(): GitHubAuth {
const auth = {
username: this.config.get('gh_username'),
password: this.config.get('gh_password'),
};
return auth;
}
private checkGithubLogin(): boolean {
if (this.config.get('gh_username') || this.config.get('gh_password')) {
return true;
}
return false;
}
/**
* Get class's singleton instance
* @param appName
*/
public static getInstance(): App {
if (!App.instance) {
App.instance = new App();
}
return App.instance;
}
/**
* Start app
*/
public start() {
this.version(this.pkg.packageJson.version);
this.description(this.pkg.packageJson.description);
this.parse(process.argv);
if (!process.argv.slice(2).length) {
this.outputHelp();
}
}
/**
* list available serial ports
*/
public async listSerialPorts() {
const loading = ora('Looking for available serial ports').start();
// create table
const table = new Table({
head: [chalk.blue('Path'), chalk.blue('Manufacturer')],
colWidths: [25, 25],
});
// get serial ports
helper.getPorts().then(
(ports: readonly Port[]) => {
// no serial port found
if (ports.length <= 0) {
loading.warn(`No serial port available`);
return;
}
// done loading
loading.succeed();
// populate table
ports.forEach((port) => {
table.push([port.path, port.manufacturer]);
});
// show table
console.log(table.toString());
},
(error) => {
loading.fail(`Could not retrieve serial ports`);
// TODO: Only show detailed error message if verbose output enabled.
console.log(chalk.red(`Error: ${error}`));
}
);
}
/**
* Get chip id
* @param port
*/
public async getChipId(port: string) {
const loading = ora();
// if no port provided as argument, prompt with available ports.
if (!port) {
port = (await prompts.selectPort()).path;
}
loading.start('Getting chip id from device');
helper.getChipId(port).then(
(chipId) => {
loading.succeed(`Chip id: ${chalk.green(chipId)}`);
},
(error) => {
loading.fail(`Could not read chip id from device`);
// TODO: Only show detailed error message if verbose output enabled.
console.log(chalk.red(`Error: ${error}`));
}
);
}
/**
* Upload firmware
*/
public async uploadFirmware(options: {
port: string;
dir: string;
lock: string;
slaves: number;
webui: boolean;
trial: number;
wizard: boolean;
}) {
const loading = ora();
// if no port provided, prompt with available ports.
if (!options.port) {
options.port = (await prompts.selectPort()).path;
}
// if no firmware directory provided, use current working directory.
if (!options.dir) {
options.dir = process.cwd();
}
loading.start('Compiling and uploading firmware');
if (!this.checkGithubLogin()) {
loading.fail(chalk.red('Github credentials not found in config'));
return;
}
const buildOptions: BuildOptions = {
chipId: options.lock ? options.lock : '',
maxSlave: options.slaves ? options.slaves : 4,
trialMode: options.trial ? true : false,
trialTime: options.trial ? options.trial : 1440,
};
helper
.buildAndUpload(
options.dir,
options.port,
this.getGithubLogin(),
buildOptions
)
.then(
() => {
loading.succeed(chalk.greenBright('Firmware successfully uploaded'));
},
(error) => {
loading.fail(
`An error has ocurred trying to build and upload firmware`
);
// TODO: Only show detailed error message if verbose output enabled.
console.log(chalk.red(`Error: ${error}`));
}
);
}
/**
* Erase device flash memory
* @param port
*/
public async eraseFlash(port: string) {
const loading = ora();
// if no port provided as argument, prompt with available ports.
if (!port) {
port = (await prompts.selectPort()).path;
}
loading.start(chalk.red('Performing flash erase on device'));
helper.eraseFlash(port).then(
() => {
loading.succeed(
chalk.greenBright('Device flash has been successfully erased')
);
},
(error) => {
loading.fail(`An error has ocurred trying to erase device flash`);
// TODO: Only show detailed error message if verbose output enabled.
console.log(chalk.red(`Error: ${error}`));
}
);
}
/**
* Show config menu
*/
public async showConfigMenu() {
prompts.configMenu().then((configMenuSelection: { config: string }) => {
switch (configMenuSelection.config) {
case 'github':
if (this.checkGithubLogin()) {
console.log(chalk.green('Github credentials already exist.'));
console.log(chalk.grey(JSON.stringify(this.getGithubLogin())));
inquirer
.prompt({
type: 'confirm',
name: 'editCredentials',
message: 'Would you like to modify them?',
default: false,
})
.then((result) => {
if (result.editCredentials) {
prompts.addGithubLogin().then((entry: GitHubAuth) => {
this.storeGithubLogin(entry.username, entry.password);
console.log(chalk.greenBright('Saved!'));
});
} else {
return;
}
});
} else {
prompts.addGithubLogin().then((entry: GitHubAuth) => {
this.storeGithubLogin(entry.username, entry.password);
});
}
break;
default:
break;
}
});
}
}
const app = App.getInstance();
export default app;
<file_sep># Changelog
All notable changes to this project will be documented in this file. See [standard-version](https://github.com/conventional-changelog/standard-version) for commit guidelines.
### 1.0.1 (2020-09-25)
### Bug Fixes
* **index:** fix commands options arguments ([3a2022b](https://github.com/iotbits-us/mbox-builder-cli/commit/3a2022b75499ad5220dae2c931108b079ea452f2))
* **index:** fix erase command options ([346baa1](https://github.com/iotbits-us/mbox-builder-cli/commit/346baa1c33e1aa9e7536d83207ebbfd4e72e1538))
<file_sep>export type Port = {
readonly path: string;
readonly manufacturer?: string;
};
<file_sep># ModbusBox Firmware Builder CLI
ModbusBox Firmware Builder CLI
<file_sep>import inquirer from 'inquirer';
import helper, { BuildOptions } from 'mbox-builder-helper';
import ora from 'ora';
import { Port } from './model';
/**
* Prompt available serial ports
* Show available serial port and return a promise with the one selected.
*/
export const selectPort = (): Promise<Port> => {
return new Promise((resolve, reject) => {
const loading = ora('Looking for available serial ports').start();
helper.getPorts().then(
(ports: readonly Port[]) => {
if (ports.length <= 0) {
loading.warn(`No serial port available`);
return;
}
loading.succeed();
inquirer
.prompt([
{
type: 'rawlist',
name: 'port',
message: 'Please select a port',
choices: ports.map((port) => {
return port.path;
}),
filter: function (val) {
return val;
},
},
])
.then((answer) => {
resolve({ path: answer.port });
});
},
(error) => {
loading.fail(`Could not retrieve serial ports`);
reject(error);
}
);
});
};
const configMenuQuestions = [
{
type: 'list',
name: 'config',
message: 'What would you like to configure?',
choices: [
{ name: 'GitHub Credentials', value: 'github' },
{ name: 'Default Building Options', value: 'building', disabled: true },
{ name: 'Debug Info', value: 'debug', disabled: true },
],
filter: function (val: string) {
return val.toLowerCase();
},
},
];
export const configMenu = () => {
return new Promise((resolve) => {
inquirer.prompt(configMenuQuestions).then((mainMenuSelection) => {
resolve(mainMenuSelection);
});
});
};
const addGithubLoginQuestions = [
{
type: 'input',
name: 'username',
message: 'Enter your Github username:',
filter: String,
},
{
type: 'password',
name: 'password',
message: 'Enter your Github password:',
},
];
export const addGithubLogin = () => {
return new Promise((resolve) => {
inquirer.prompt(addGithubLoginQuestions).then((answers) => {
resolve(answers);
});
});
};
const uploadWizardQuestions = [
{
type: 'confirm',
name: 'lockToDevice',
message: 'Would you like to lock the firmware to the device on USB port?',
default: false,
},
{
type: 'input',
name: 'slaves',
message: 'Enter the maximum amount of slaves 1-4:',
validate: function (value: string) {
const valid =
!isNaN(parseInt(value)) && parseInt(value) > 0 && parseInt(value) < 5;
return valid || 'Please enter a number between 1 and 4';
},
filter: Number,
},
{
type: 'input',
name: 'trialTime',
message: 'Enter the trial time:',
validate: function (value: string) {
const valid = !isNaN(parseInt(value));
return valid || 'Please enter a number';
},
filter: Number,
},
];
export const uploadWizard = (): Promise<BuildOptions> => {
return new Promise((resolve) => {
const buildOptions: BuildOptions = {};
inquirer.prompt(uploadWizardQuestions).then((answers) => {
console.log(answers);
resolve(buildOptions);
});
});
};
| d9027e8adc771ee33fce7393df1cc658a231bb7b | [
"Markdown",
"TypeScript"
] | 6 | TypeScript | iotbits-us/mbox-builder-cli | 4204ea17e3790fc7229f8b53898b0f4920692611 | 7a169c6ae15ee849017f910ed20f8fec3486fe97 | |
refs/heads/master | <repo_name>bubbabjh/cp1<file_sep>/mjs.js
var x = 0;
var fans = 0;
var cpc = 1;
var footballs = 0;
var missionaries = 0;
var cpcCost = 25;
var fBCost = 50;
var mishCost = 300;
function test(event)
{
x += cpc;
document.getElementById("numclicks").innerHTML = x + (x ==1 ? " Provo point" : " Provo points");
event.preventDefault();
}
function darken(event)
{
document.getElementById("btn").src = "./Yclick2.png";
}
function lighten(event)
{
document.getElementById("btn").src = "./Yclick1.png";
}
function addTC(event)
{
if ((x >= cpcCost))
{
x -= cpcCost;
cpcCost = Math.ceil(cpcCost*1.4);
cpc += 1;
}
document.getElementById("costcoug").innerHTML = "Buy one cougar for " + cpcCost + " Provo points (+1Pppc)";
document.getElementById("numclicks").innerHTML = x + (x ==1 ? " click" : " Provo points");
}
function addFB(event)
{
if ((x >= fBCost))
{
x -= fBCost;
fBCost = Math.ceil(fBCost *= 1.5);
footballs += 1;
}
document.getElementById("costFB").innerHTML = "Buy one football for " + fBCost + " Provo points (+1Ppps)";
document.getElementById("numclicks").innerHTML = x + (x ==1 ? " click" : " Provo points");
}
function addMish(event)
{
if ((x >= mishCost))
{
x -= mishCost;
mishCost = Math.ceil(mishCost *= 1.4);
missionaries += 1;
}
document.getElementById("costMish").innerHTML = "Buy one missionary for " + mishCost + " Provo points (+5Ppps)";
document.getElementById("numclicks").innerHTML = x + (x ==1 ? " click" : " Provo points");
}
function winGame(event)
{
if ((x >= 400))
{
x -= 400;
fans += 1;
document.getElementById("fans").innerHTML = fans + (fans ==1 ? " Fan" : " fans");
}
}
function addPS()
{
x += Math.ceil(footballs + footballs*.2*fans);
x += missionaries * 5;
document.getElementById("numclicks").innerHTML = x + (x ==1 ? " Provo point" : " Provo points");
}
function getStatus()
{
if (x < 200)
{
document.getElementById("statuss").innerHTML = "You're an incoming freshman at UVU";
}
else if (x < 500)
{
document.getElementById("statuss").innerHTML = "You downloaded mutual";
}
else if (x < 1000)
{
document.getElementById("statuss").innerHTML = "You've successfuly transfered to BYU";
}
else if (x < 5000)
{
document.getElementById("statuss").innerHTML = "People have started calling you a \"Provo all-star\"";
}
else if (x < 10000)
{
document.getElementById("statuss").innerHTML = "You got into your program of choice";
}
else if (x < 15000)
{
document.getElementById("statuss").innerHTML = "You've met your soulmate";
}
else if (x < 25000)
{
document.getElementById("statuss").innerHTML = "You've gratuated, Congratulations!";
}
else
{
document.getElementById("statuss").innerHTML = "Your kids have been accepted into BYU";
}
event.preventDefault();
}
function constant()
{
setInterval('addPS(); getStatus();', 1000);
}
| fcf6afa5fcca3b161384fbd009454fb9b88452fa | [
"JavaScript"
] | 1 | JavaScript | bubbabjh/cp1 | ce99880bdc252f06aff5347cc52fffbdae0ad471 | 0a6aef9b0549fca1cb1a42e86bc851fb98dcf734 | |
refs/heads/main | <repo_name>lihongtang95/streamlit_wsb_app<file_sep>/README.md
# streamlit_wsb_app
A Streamlit/Heroku web application that pulls comments from the most recent Daily Discussion forums of the WallStreetBets subreddit and provides a visual summary of the most-mentioned stock tickers. The link is below.
NOTE: The heroku app can take a couple minutes to load. The number of comments per pushshift request is limited, and larger discussion threads can have upwards of 20,000 comments. Working on improving this.
https://wsb-daily-trending-app.herokuapp.com/
<file_sep>/requirements.txt
streamlit==0.87.0
pandas==1.0.5
numpy==1.19.5
selenium==3.141.0
requests==2.21.0
yfinance==0.1.63
plotly==4.10.0<file_sep>/WSB_app.py
import streamlit as st
from selenium import webdriver
import os
from collections import Counter
from datetime import date,timedelta
from dateutil.parser import parse
import requests
import time
import pandas as pd
import numpy as np
import yfinance as yf
import plotly.graph_objects as go
from PIL import Image
# DATALAG default to 0
# If PushShift is behind, set to more than 0
DATALAG = 1
# Create a webdriver to navigate to WSB subreddit
def grab_html():
#If today is MON 0 or SUN 6 then we get data from a weekend discussion thread. Else, daily.
global DATALAG
dateoffset = date.today().weekday() - DATALAG
days = [0,1,2,3,4,5,6]
if days[dateoffset] in (1,2,3,4,5):
url = 'https://www.reddit.com/r/wallstreetbets/search/?q=flair%3A%22Daily%20Discussion%22&restrict_sr=1&sort=new%27'
else:
url = 'https://www.reddit.com/r/wallstreetbets/search/?q=flair%3A%22Weekend%20Discussion%22&restrict_sr=1&sort=new%27'
CHROMEDRIVER_PATH = "/app/.chromedriver/bin/chromedriver"
chrome_bin = os.environ.get('GOOGLE_CHROME_BIN', 'chromedriver')
options = webdriver.ChromeOptions()
options.binary_location = chrome_bin
options.add_argument('--disable-gpu')
options.add_argument('--no-sandbox')
options.add_argument('--headless')
browser = webdriver.Chrome(executable_path=CHROMEDRIVER_PATH, chrome_options=options)
browser.get(url)
return browser
# Getting the post ID of the daily or weekend thread to use with PushShift later
def grab_link(driver):
global DATALAG
links = driver.find_elements_by_xpath('//*[@class="_eYtD2XCVieq6emjKBH3m"]')
for a in links:
if a.text.startswith('Daily Discussion Thread'):
yesterday_sub_lag = date.today() - timedelta(days=1+DATALAG)
DATE = ''.join(a.text.split(' ')[-3:])
parsed = parse(DATE)
if parse(str(yesterday_sub_lag)) == parsed:
link = a.find_element_by_xpath('../..').get_attribute('href')
stock_link = link.split('/')[-3]
driver.close()
return stock_link, link
if a.text.startswith('Weekend'):
if (date.today() - timedelta(days=DATALAG)).weekday() == 0:
friday = date.today() - timedelta(days=3+DATALAG)
elif (date.today() - timedelta(days=DATALAG)).weekday() == 6:
friday = date.today() - timedelta(days=2+DATALAG)
else:
print('uh oh!')
DATE = ''.join(a.text.split(' ')[-3:])
parsed = parse(DATE)
if parse(str(friday)) == parsed:
link = a.find_element_by_xpath('../..').get_attribute('href')
stock_link = link.split('/')[-3]
driver.close()
return stock_link, link
# Get list of comment IDs to search
def grab_commentid_list(stock_link):
html = requests.get(f'https://api.pushshift.io/reddit/submission/comment_ids/{stock_link}')
raw_comment_list = html.json()
return raw_comment_list
# Prepare list of stocks to cross-reference with the comments
def grab_stocklist():
stocklist = []
with open('REDDIT_STOCK_LIST.txt', 'r') as w:
stocks = w.readlines()
for a in stocks:
a = a.replace('\n','').replace('\t','')
stocklist.append(a)
#stocks_list = ['BABA','MSFT','SOFI','MVST','AMC','GME','AMZN','CLOV','BB','SPY','MRNA','QQQ']
# Remove common English words from list of tickers
blacklist = ['I','B','R','L','C','A','DD','PT','MY','ME','FOR','EOD','GO','TA','USA','AI','ALL','ARE','ON','IT','F','SO','NOW','REE','CEO']
#greylist = []
for stock in blacklist:
stocklist.remove(stock)
# for stock in greylist:
# stocklist.remove(stock)
return stocklist
# Use comment IDs to get comment text
def get_comments(comment_list):
comment_list = comment_list['data']
l = comment_list.copy()
string = ''
html_list = []
# Request size upper limit ranges between 500-700. Will retry a request if failed
# def make_request(uri, max_retries = 5):
# def fire_away(uri):
# response = requests.get(uri)
# print('STATUS:', response.status_code)
# assert response.status_code == 200
# return response
# current_tries = 0
# while current_tries < max_retries:
# try:
# time.sleep(1)
# response = fire_away(uri)
# return response
# except:
# time.sleep(1)
# current_tries += 1
# return fire_away(uri)
for i in range(1,len(comment_list)+1):
string += l.pop() + ','
if i % 450 == 0:
html = requests.get(f'https://api.pushshift.io/reddit/comment/search?ids={string}&fields=body')
html_list.append(html.json())
string = ''
#Getting last chunk leftover, not divisible by request size
if string:
html = requests.get(f'https://api.pushshift.io/reddit/comment/search?ids={string}&fields=body')
html_list.append(html.json())
return html_list
# Count a stock if it matches a ticker in stock list
def get_stock_list(newcomments,stocks_list):
stock_dict = Counter()
for chunk in newcomments:
for a in chunk['data']:
for ticker in stocks_list:
parsedTicker1 = '$' + ticker + ' '
parsedTicker2 = ' ' + ticker + ' '
#parsedTicker3 = ' ' + ticker.lower().capitalize() + ' '
if parsedTicker1 in a['body'] or parsedTicker2 in a['body']: #or parsedTicker3 in a['body']:
stock_dict[ticker]+=1
return stock_dict
# Do everything
if __name__ == "__main__":
driver = grab_html()
stock_link, link = grab_link(driver)
comment_list = grab_commentid_list(stock_link)
stocks_list = grab_stocklist()
new_comments = get_comments(comment_list)
stock_dict = get_stock_list(new_comments,stocks_list)
#stock_dict = {'HOOD': 62, 'NVDA': 59, 'TSLA': 58, 'PFE': 52, 'TLRY': 33, 'AMD': 32, 'BABA': 11}
df = pd.DataFrame()
df['ticker'] = stock_dict.keys()
df['mentions'] = stock_dict.values()
df = df.sort_values(by=['mentions'], ascending=False, ignore_index=True)
df = df.head(10)
# Putting everything together
image = Image.open('wsb.jpeg')
col1, col2, col3 = st.columns([1.5,6,1])
with col1:
pass
with col2:
st.image(image)
with col3:
pass
st.write("###")
st.title(f'WSB Daily Trending Stocks for {date.today().strftime("%m/%d/%Y")}')
st.write("#")
url= link
st.markdown(url, unsafe_allow_html=True)
st.write("###")
col1, col2, col3 = st.columns([2.5,6,1])
with col1:
pass
with col2:
st.write(df)
with col3:
pass
st.write("#")
df = df.head(5)
for index, row in df.iterrows():
ticker = row['ticker']
st.write(f"""
# ${ticker}
""")
tickerSymbol = ticker
tickerData = yf.Ticker(tickerSymbol)
# Get the 30 day price history for ticker
tickerDf = tickerData.history(start=date.today() - timedelta(days=30), end=date.today())
tickerDf = tickerDf.reset_index()
for i in ['Open', 'High', 'Close', 'Low']:
tickerDf[i] = tickerDf[i].astype('float64')
fig = go.Figure(data=[go.Candlestick(x=tickerDf['Date'],
open=tickerDf['Open'],
high=tickerDf['High'],
low=tickerDf['Low'],
close=tickerDf['Close'])])
fig.update_layout(height=650,width=900)
st.plotly_chart(fig, use_container_width=True)
col1, col2, col3 = st.columns([1,6,1])
with col1:
pass
with col2:
st.line_chart(tickerDf.Volume, use_container_width=True)
with col3:
pass
st.write("##")
| 34e73f06daee68985851a6a6b5c37eec64d24f96 | [
"Markdown",
"Python",
"Text"
] | 3 | Markdown | lihongtang95/streamlit_wsb_app | 4e74bc1d458d9dd028942532078310cebef9417b | 35140e5701bd8f0722c3095d66735417ee51f60b | |
refs/heads/main | <repo_name>kartikbariya11/textutils<file_sep>/src/components/TextForm.js
import React, { useState } from "react";
export default function TextForm(props) {
const handleUpClick = ()=>{
// console.log("clicked");
let newText = text.toUpperCase();
setText(newText);
props.showAlert("converted to upperCase","success");
};
const handleLowClick = ()=>{
// console.log("clicked");
let newText = text.toLowerCase();
setText(newText);
props.showAlert("converted to lowerCase","success");
};
const handleReverseClick = ()=>{
// console.log("clicked");
let newText = text.split("").reverse().join("");
setText(newText);
props.showAlert("Text Reversed","success");
};
const handleOnChange = (event)=>{
// console.log("handleUpChange");
setText(event.target.value);
};
const [text, setText] = useState("");
return (
<>
<div className="container" style={{color: props.mode==='dark'?'white':'#042743'}}>
<h1 >{props.heading}</h1>
<div className="mb-3">
<textarea className="form-control" value={text} onChange={handleOnChange}
style={{backgroundColor: props.mode==='dark'?'grey':'white', color : props.mode==='dark'?'white':'#042743'}}
id="myBox" rows="8"></textarea>
</div>
<button className="btn btn-primary mx-2" onClick={handleUpClick}
// style={{backgroundColor: props.mode==='dark'?'#235600':'blue'}}
>
Convert To Uppercase
</button>
<button className="btn btn-primary mx-2" onClick={handleLowClick}
// style={{backgroundColor: props.mode==='dark'?'#235600':'blue'}}
>
Convert To Lowercase
</button>
<button className="btn btn-primary mx-2" onClick={handleReverseClick}
// style={{backgroundColor: props.mode==='dark'?'#235600':'blue'}}
>
Reverse
</button>
</div>
<div className="container my-2" style={{color: props.mode==='dark'?'white':'#042743'}}>
<h1>Your text summary</h1>
<p>Words{text.length>0 ? text.trimStart().trimEnd().split(" ").length : 0} ,charaxcters {text.length}</p>
<p> {0.008 * text.split(" ").length } time min</p>
<h1>Preview</h1>
<p>{text.length>0?text:"enter something to preview it."}</p>
</div>
</>
);
}
| 16807a534103f5e03307956b80fb97229af4ae59 | [
"JavaScript"
] | 1 | JavaScript | kartikbariya11/textutils | eb2d9a1f8214b109f795a36c7aebe4f266f2e4d0 | f1f7f0c09f8646c363cd61643b8f870349aa1341 | |
refs/heads/master | <file_sep># MediaDb
MediaDb — theme for UiB's MediaDb.
## About
Based on the [blank](http://themes.gohugo.io/theme/blank/) theme.
## License
This theme is released under the [MIT license](//github.com/Vimux/blank/blob/master/LICENSE.md).
<file_sep>name = "MediaDb"
license = "MIT"
licenselink = "https://github.com/vimux/blank/blob/master/LICENSE.md"
description = "MediaDb — theme for UiB's MediaDb."
homepage = "https://github.com/semafor/blank/"
tags = []
features = ["blog"]
min_version = 0.15
[author]
name = "Vimux"
homepage = "https://github.com/vimux"
<file_sep>window.addEventListener("load", function load(event) {
var container = document.getElementById("all-movies-placeholder");
if (!container) {
return;
}
var tmpl = mediadb.templates.movie_summary;
for (var movie_id in movies_object) {
movies_object[movie_id]._key = movie_id;
movies_object[movie_id].art_path = getNelsonImageUrl(movie_id);
container.innerHTML += template(tmpl, movies_object[movie_id]);
}
});
<file_sep>function toggleSiteNav() {
document.getElementById("main-nav").classList.toggle("open");
}
<file_sep>function openDialog() {
document.body.classList.add("show-dialog");
}
function closeDialog() {
document.body.classList.remove("show-dialog");
}
<file_sep>window.addEventListener("load", function load(event) {
var placeholder = document.getElementById("single-genre-placeholder");
if (!placeholder) {
return;
}
var query_params = get_query_string_parameters(document.location.href);
var genre = query_params.title;
var movies = [];
console.log(genres_object)
for (var movieId in genres_object) {
for (var genreName in genres_object[movieId]) {
if (genreName.indexOf(genre) >= 0) {
movies.push(movieId);
}
}
}
var tmp = document.createElement('div');
tmp.innerHTML = template(
mediadb.templates.genre_single, { title: genre }
);
placeholder.parentNode.insertBefore(tmp, placeholder);
render_set_of_movies("single-genre-placeholder", movies);
});
<file_sep>---
made:
actors:
- <NAME>
- <NAME>
alternateTitles:
nb_no:
genres:
- horror
- suspense
summary: ""
extraMaterial:
- deleted scenes
- gaffes
runtime: 0
originCountries: []
type: film
artwork: []
---
<file_sep>{{ partial "header.html" . }}
<main class="genre-single single-view">
<article>
<h1>{{ .Title }}</h1>
<h2>Filmer i denne sjangeren:</h2>
<ul class="film-list">
{{ range .Params.associatedFilms }}
{{ range . }}
<li><a href="{{ "/film/" | relLangURL }}{{ .url | urlize }}.html">{{ .name }}</a> </li>
{{ end }}
{{ end }}
</ul>
</article>
</main>
{{ partial "footer.html" . }}
<file_sep>function search_for_movie(keyword, movies) {
return movies.filter(
m => (m.ntitle.toLowerCase().indexOf(keyword.toLowerCase()) >= 0) ||
(m.otitle.toLowerCase().indexOf(keyword.toLowerCase()) >= 0)
);
}
function search_for_genre(genre, movies) {
return movies.filter(function (m) {
for (var genreName in genres_object[m._key]) {
if (genreName.indexOf(genre.toLowerCase()) >= 0) {
return true;
}
}
return false;
});
}
function search_for_cast(cast, movies) {
return movies.filter(function (m) {
if (!m.folk) {
return false;
}
var folk = m.folk.split(", ");
for (var i = 0; i < folk.length; i++) {
var f = folk[i].toLowerCase().trim();
if (f.indexOf(cast) >= 0) {
return true;
}
}
return false;
});
}
function display_search_for_movie(results, container) {
if (results.length == 0) {
container.innerHTML = mediadb.templates.no_results;
return;
}
var tmpl = mediadb.templates.movie_summary;
container.innerHTML = "<div class='movie-section front-section'>";
for (var i = 0; i < results.length; i++) {
results[i].art_path = getNelsonImageUrl(results[i]._key);
container.innerHTML += template(tmpl, results[i]);
}
container.innerHTML += "</div>";
}
window.addEventListener("load", function load(event) {
// Whether or not to do a search.
var placeholder = document.getElementById("search-results-placeholder");
if (!placeholder) {
return;
}
query_params = get_query_string_parameters(
document.location.href.toLowerCase()
);
// Create an array of movie objects and preserve the key.
search_results = [];
for (var key in movies_object) {
var obj = movies_object[key];
obj["_key"] = key;
search_results.push(obj);
}
if (query_params.cast) {
var cast = query_params.cast.split(" ");
for (var i = 0; i < cast.length; i++) {
search_results = search_for_cast(cast[i], search_results);
}
}
if (query_params.director) {
search_results = search_results.filter(
m => (m.dir && m.dir.toLowerCase().indexOf(query_params.director) >= 0)
);
}
if (query_params.genre) {
var genres = query_params.genre.split(",");
for (var i = 0; i < genres.length; i++) {
search_results = search_for_genre(genres[i].trim(), search_results);
}
}
if (query_params.country) {
search_results = search_results.filter(
m => (m.dir && m.country.toLowerCase() == query_params.country)
);
}
if (query_params.search) {
search_results = search_for_movie(query_params.search, search_results);
}
display_search_for_movie(search_results, placeholder);
});
<file_sep>---
name: ""
films:
- intouchables
---
<file_sep>window.addEventListener("load", function load(event) {
var container = document.getElementById("single-movie-placeholder");
if (!container) {
return;
}
var query_params = get_query_string_parameters(document.location.href);
if (!query_params.id) {
window.location.href = "/404.html";
return;
}
var xhr = new XMLHttpRequest();
xhr.open("GET", "http://wildboy.uib.no/mongodb/objects/?filter_id=" + query_params.id, true);
xhr.responseType = "json";
xhr.onload = function () {
movie_object = xhr.response.rows[0];
if (!movie_object) {
window.location.href = "/404.html";
return;
}
// get the genre info (if it exists)
var genre_object = genres_object[query_params.id];
// get the review info (if it exists)
var review_object = reviews_object[query_params.id];
// Slap some more stuff onto the movie_object
movie_object.art_path = getNelsonImageUrl(query_params.id);
// Genre stuffs.
movie_object.genre_html = "<nav class='genre-nav inline-menu'><ul>";
for (var k in genre_object) {
movie_object.genre_html += template(mediadb.templates.genre, {
title: k
});
}
movie_object.genre_html += "</ul></nav>";
// People stuff.
movie_object.people_html = "<ul class='actor-list'>";
var people_list = movie_object.folk ? movie_object.folk.split(",") : [];
for (var i = 0; i < people_list.length; i++) {
movie_object.people_html += template(mediadb.templates.person, {
title: people_list[i].trim()
});
}
movie_object.people_html += "</ul>";
// Trailer stuff.
movie_object.trailer_html = "";
if (movie_object["youtube trailer id"]) {
movie_object.trailer_html = template(mediadb.templates.trailer, {
youtube_embed: getYouTubeEmbed(movie_object["youtube trailer id"])
});
}
// Set up reviews html.
if (review_object) {
movie_object.reviews_html = "<ul class='reviews'>";
var avg = 0;
var numReviews = 0;
var reviews = "";
for (var reviewId in review_object) {
avg += review_object[reviewId].rating;
numReviews++;
reviews += template(mediadb.templates.review, review_object[reviewId]);
}
if (numReviews) {
var tmpl = mediadb.templates.rating_average;
avg = avg / numReviews;
avg = avg.toFixed(1);
movie_object.reviews_html += template(tmpl, {'avg': avg});
}
movie_object.reviews_html += reviews;
movie_object.reviews_html += "</ul>";
}
container.innerHTML = template(mediadb.templates.movie_single, movie_object);
}
xhr.send();
});
| c0416f5b2aba1a4de5ab98492706a85e40c354d1 | [
"Markdown",
"TOML",
"JavaScript",
"HTML"
] | 11 | Markdown | semafor/blank | 5aac3ad30b75dc3303c514adeebe2cbb4addcbb2 | a7d4faf5f731264e95cd207056a6876096a2f546 | |
refs/heads/master | <file_sep>import { Injectable } from '@angular/core';
import 'rxjs/add/operator/map';
import * as faker from 'faker';
/*
Generated class for the Agenda provider.
See https://angular.io/docs/ts/latest/guide/dependency-injection.html
for more info on providers and Angular 2 DI.
*/
@Injectable()
export class Agenda {
constructor() {}
getContacts():Contact[]{
let contacts = [];
for(let i=0; i< 500; i++){
let contact:Contact ={
name:faker.name.findName(),
phone:faker.phone.phoneNumber(),
imgUrl: faker.image.avatar()
}
contacts.push(contact);
}
contacts.sort((a:Contact, b:Contact)=>{
if (a.name < b.name)
return -1;
if(a.name > b.name)
return 1;
else return 0;
});
return contacts;
}
}
export interface Contact{
name:string,
phone:number,
imgUrl:string
}
<file_sep># Ionic 3 Virtual Scroll example
This project is an example showing how to use Ionic 3 virtual scroll feature, with custom header dividers.
For more info, visit the blog post about this code: [http://blog.enriqueoriol.com/2017/06/ionic-3-virtual-scroll.html](http://blog.enriqueoriol.com/2017/06/ionic-3-virtual-scroll.html)
## Install
Execute in terminal `npm install`
If you don't have ionic CLI installed, run also `npm install -g ionic`
## Run
Execute in terminal `ionic serve`
<file_sep>import { Component, OnInit } from '@angular/core';
import { Agenda, Contact } from "../../providers/agenda";
@Component({
selector: 'page-contacts',
templateUrl: 'contacts.html'
})
export class ContactsPage implements OnInit {
public items:Array<Contact> = new Array<Contact>();
constructor(public agenda: Agenda) {
}
ngOnInit(){
this.items = this.agenda.getContacts();
}
checkInitialChange(item, itemIndex, items){
if(itemIndex == 0)
return item.name[0];
if(item.name[0] != items[itemIndex-1].name[0])
return item.name[0];
return null;
}
}
| a004005b873497aed6a8a8de6dc1a0111d600f7c | [
"Markdown",
"TypeScript"
] | 3 | TypeScript | kaikcreator/ionicVirtualScroll | e87afde4d3c07eb78a6b411044e899f43dd496c6 | 0feb26c8c0115d63e1e314890f0a122283322da5 | |
refs/heads/master | <repo_name>rhiannanberry/Seven-Dancing-Ghost-Simulators<file_sep>/Assets/Scripts/PlayerRaycast.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerRaycast : MonoBehaviour {
[SerializeField]
private Camera cam;
[SerializeField]
private float scale = 2f;
public bool lockCursor = false;
public DDRCube ddrCube;
// Use this for initialization
// Update is called once per frame
void Start ()
{
}
void FixedUpdate () {
if (Input.GetMouseButtonDown(0))
{
lockCursor = DetectHit();
}
}
private bool DetectHit()
{
RaycastHit hit;
Ray ray = new Ray(cam.transform.position, cam.transform.forward);
if (Physics.Raycast(ray, out hit))
{
DDRCube dCopy = Instantiate<DDRCube>(ddrCube);
dCopy.transform.rotation = transform.rotation;
dCopy.setDestination(hit.point);
return true;
}
return false;
}
}
<file_sep>/Assets/Scripts/Arrow.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Arrow : MonoBehaviour {
private Vector3 destination;
private bool destHit = false;
private Vector3 direction;
private Vector3 startPos;
private KeyCode arrowDir;
private bool destArr = false;
[SerializeField]
private float time = 2f; //this should be TIME it takes to get to destination so all arrows match on all screen ratios
[SerializeField]
private float speed;
private Material alt;
// Use this for initialization
void Start () {
startPos = transform.position;
}
public void setDestination(Vector3 dest)
{
destination = dest;
direction = destination - transform.position;
speed = direction.magnitude / time;
direction = direction.normalized;
}
public void setRotation(Quaternion adj)
{
transform.rotation = Camera.main.transform.rotation * adj;
}
public void setDestAndDir(bool val, KeyCode dir)
{
arrowDir = dir;
destArr = val;
if (val)
{
GetComponent<Renderer>().material = alt;
}
}
public void resetPosition()
{
transform.position = startPos;
destHit = false;
}
// Update is called once per frame
void FixedUpdate () {
if (!destArr)
{
if (transform.position == destination || destHit)
{
destHit = true;
transform.Translate(direction * Time.deltaTime * speed, Space.World);
//transform.position = startPos;
} else
{
transform.position = Vector3.MoveTowards(transform.position, destination, Time.deltaTime * speed);
}
}
}
void OnTriggerStay(Collider collider)
{
if (destArr && collider.tag == "Arrow")
{
if (Input.GetKeyDown(arrowDir))
{
Debug.Log(arrowDir);
(collider.GetComponentInParent<Arrow>()).resetPosition();
}
}
}
}
<file_sep>/Assets/Scripts/Line.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Line : MonoBehaviour {
public LineRenderer lr;
List<Vector3> points;
public void UpdateLine(Vector3 mousePos) {
if (points == null) {
points = new List<Vector3>();
SetPoint(mousePos);
return;
}
if (Vector2.Distance(points[points.Count-1], mousePos) > .03f) {
SetPoint(mousePos);
}
}
void SetPoint (Vector3 mousePos) {
points.Add(mousePos);
lr.positionCount = points.Count;
lr.SetPosition(points.Count - 1, mousePos);
}
}
<file_sep>/Assets/Scripts/Snowflake.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Snowflake : MonoBehaviour {
Camera sourceCam;
// Use this for initialization
void Start () {
sourceCam = transform.parent.GetComponent<Camera>();
sourceCam.aspect = 1;
float sideLenPixels = sourceCam.scaledPixelWidth;
Vector3 start = sourceCam.ScreenToWorldPoint(new Vector2(0,0));
Vector3 end = sourceCam.ScreenToWorldPoint(new Vector2(sideLenPixels, 0));
float worldSize = Vector3.Distance(start, end)/4;
//transform.SetParent(null);
transform.localScale = Vector3.one * worldSize;
//transform.SetParent(sourceCam.transform);
Vector3 pos = Camera.main.ScreenToWorldPoint(new Vector3(Screen.width/2, Screen.height/2, Camera.main.nearClipPlane+1.5f));
transform.position = pos;//new Vector3(transform.position.x, transform.position.y, pos.z);
transform.localPosition = new Vector3(-1, 1, transform.localPosition.z);
}
// Update is called once per frame
void Update () {
}
}
<file_sep>/Assets/Scripts/SongController.cs
using System.Collections;
using System.Collections.Generic;
using System.IO;
using UnityEngine;
public class SongController : MonoBehaviour {
public struct Metadata {
public bool valid;
public string title;
public string subtitle;
public string artist;
public string musicPath; //gonna use resources load for this probably like Resources.Load("music/0000")
//likely will be necessary to import the succes and failure sounds as well
public float offset;
public float bpm;
public NoteData noteData;
}
public struct NoteData {
public List<List<NoteData>> bars;
}
public struct Notes {
public bool left;
public bool right;
public bool up;
public bool down;
}
// Use this for initialization
void Start () {
}
void setSong(string songName) {
//TODO: introduce error handling for invalid sm
bool inNotes = false; //are we parsing info metadata or notes
Metadata songData = new Metadata();
songData.valid = true;
songData.musicPath = songName; //does this need extension or?
string[] fileData = File.ReadAllLines("SMFiles/" + songName + ".sm");
foreach (string line in fileData) {
if (line.StartsWith("//")) {
continue;
} else if (!inNotes && line.StartsWith("#")) {
string key = line.Substring(0, line.IndexOf(":")).Trim('#').Trim(':');
switch (key.ToUpper()) {
case "TITLE":
songData.title = line.Substring(line.IndexOf(':')).Trim(':').Trim(';');
break;
case "SUBTITLE":
songData.subtitle = line.Substring(line.IndexOf(':')).Trim(':').Trim(';');
break;
case "OFFSET":
if (!float.TryParse(line.Substring(line.IndexOf(':')).Trim(':').Trim(';'), out songData.offset)) {
//Error Parsing
songData.offset = 0.0f;
}
break;
case "DISPLAYBPM":
if (!float.TryParse(line.Substring(line.IndexOf(':')).Trim(':').Trim(';'), out songData.bpm) || songData.bpm == 0) {
//Error Parsing - BPM not valid
songData.valid = false;
songData.bpm = 0.0f;
}
break;
case "NOTES":
inNotes = true;
break;
default:
break;
}
}
if (inNotes)
{
}
}
}
// Update is called once per frame
void Update () {
}
}
<file_sep>/Assets/Room Building/Baseboard.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Baseboard : MonoBehaviour {
Wall wall;
MeshFilter meshFilter;
Mesh prefabMesh;
Mesh mesh;
public void UpdateBaseboard() {
/*
*
* Get relevant values and create necessary arrays
*
*/
wall = GetComponentInParent<Wall>();
prefabMesh = wall.baseboard.GetComponent<MeshFilter>().sharedMesh;
meshFilter = GetComponent<MeshFilter>();
mesh = meshFilter.sharedMesh;
int prefVertexCount = prefabMesh.vertexCount;
Vector3[] prefVertices = prefabMesh.vertices; //sillohuette of the baseboard
int meshVertexCount = prefVertexCount*2;
Vector3[] meshVertices = new Vector3[meshVertexCount];
Vector2[] meshUVs = new Vector2[meshVertexCount];
int endCapTriCount = prefVertexCount-2;
int tubeTriCount = 2*(prefVertexCount-1);
int triCount = 2*endCapTriCount + tubeTriCount;
int triVertexCount = triCount*3;
int[] meshTriangles = new int[triVertexCount];
int additionalLoopCount = 1 + wall.doors.Length * 2;
if (mesh == null) {
mesh = new Mesh();
meshFilter.mesh = mesh;
}
/*
* Update vertex array and UV array
*/
//translation matrix just moves indiv. vertices like transform.Translate() does
Matrix4x4 transMatrix = Matrix4x4.Translate(Vector3.right*wall.width);
for (int i = 0; i < prefVertexCount; i++) {
Vector3 translation = transMatrix.MultiplyPoint3x4(prefVertices[i]);
meshVertices[i] = prefVertices[i];
meshVertices[i+prefVertexCount] = translation;
meshUVs[i] = prefVertices[i];
meshUVs[i+prefVertexCount] = translation;
}
/*
* Update triangle vertex array
*/
int currentTriVertex = 0;
//left end cap
for (int j = 0; j < prefVertexCount - 2; j++) {
meshTriangles[currentTriVertex++] = j+1;
meshTriangles[currentTriVertex++] = 0;
meshTriangles[currentTriVertex++] = j+2;
}
//tube
for (int k = 0; k < prefVertexCount-1; k++) {
//back triangle
meshTriangles[currentTriVertex++] = k + prefVertexCount;
meshTriangles[currentTriVertex++] = k;
meshTriangles[currentTriVertex++] = k + 1;
//front triangle
meshTriangles[currentTriVertex++] = k + prefVertexCount;
meshTriangles[currentTriVertex++] = k + 1;
meshTriangles[currentTriVertex++] = k + prefVertexCount + 1;
}
//right end cap
for (int l = prefVertexCount; l < meshVertexCount-2; l++) {
meshTriangles[currentTriVertex++] = 0;
meshTriangles[currentTriVertex++] = l+1;
meshTriangles[currentTriVertex++] = l+2;
}
/*
* Set all new mesh values and calculate normals
*/
mesh.vertices = meshVertices;
mesh.triangles = meshTriangles;
mesh.uv = meshUVs;
mesh.RecalculateNormals();
Debugging();
}
private void GenerateVerticesAndUV() {
Matrix4x4[] transMatrices = new Matrix4x4[additionalLoopCount];
int loopCnt = 0;
for (int m = 0; m < wall.doors.Length; m++) {
transMatrices[loopCnt++] = Matrix4x4.Translate(Vector3.right*wall.doors[m].transform.localPosition.x);
transMatrices[loopCnt++] = Matrix4x4.Translate(Vector3.right*(wall.doors[m].transform.localPosition.x + wall.doors[m].width));
}
transMatrices[loopCnt++] = Matrix4x4.Translate(Vector3.right*(wall.width));
for (int i = 0; i < prefVertexCount; i++) {
meshVertices[i] = prefVertices[i];
meshUVs[i] = prefVertices[i];
int transCnt = 0;
foreach (Matrix4x4 matrix in transMatrices) {
transCnt += prefVertexCount;
Vector3 translation = matrix.MultiplyPoint3x4(prefVertices[i]);
meshVertices[i + transCnt] = translation;
meshUVs[i+ transCnt] = translation;
}
}
}
private void Debugging() {
Debug.Log("DOOR BOTTOM LEFT: " + wall.doors[0].transform.localPosition.x);
Debug.Log("DOOR BOTTOM RIGHT: " + wall.doors[0].transform.localPosition.x + wall.doors[0].width);
Debug.DrawLine(Vector3.down, Vector3.right*(wall.doors[0].transform.localPosition.x + wall.doors[0].width)*wall.transform.localScale.x, Color.red, 0);
}
}
<file_sep>/Assets/Scripts/PlayerController.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerController : MonoBehaviour
{
private float speed = 5f;
private float lookSensitivity = 3f;
private PlayerDriver driver;
private PlayerRaycast pRaycast;
// Use this for initialization
void Start()
{
driver = GetComponent<PlayerDriver>();
pRaycast = GetComponent<PlayerRaycast>();
}
// Update is called once per frame
void Update()
{
if (pRaycast.lockCursor)
{
if(Cursor.lockState != CursorLockMode.None)
{
Cursor.lockState = CursorLockMode.None;
}
driver.Move(Vector3.zero);
driver.Rotate(Vector3.zero);
driver.RotateCamera(0f);
return;
}
float xMove = Input.GetAxis("Horizontal");
float zMove = Input.GetAxis("Vertical");
Vector3 horiMove = transform.right * xMove;
Vector3 vertMove = transform.forward * zMove;
Vector3 fVelocity = (horiMove + vertMove) * speed;
driver.Move(fVelocity);
//spins the actual character
float yRot = Input.GetAxisRaw("Mouse X");
Vector3 rotation = new Vector3(0f, yRot, 0f) * lookSensitivity;
driver.Rotate(rotation);
//just moves the camera up or down
float xRot = Input.GetAxisRaw("Mouse Y");
float camRotationX = xRot * lookSensitivity;
driver.RotateCamera(camRotationX);
}
}
<file_sep>/Assets/Scripts/DDRCube.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class DDRCube : MonoBehaviour {
private Camera cam;
private Vector3 startUp, startLeft, startDown, startRight;
private Quaternion rotUp, rotDown, rotLeft, rotRight;
public Arrow arrow;
public Vector3 destination;
// Use this for initialization
void Start () {
cam = Camera.main;
rotUp = Quaternion.AngleAxis(180f, Vector3.forward);
rotDown = Quaternion.AngleAxis(0f, Vector3.forward);
rotLeft = Quaternion.AngleAxis(-90f, Vector3.forward);
rotRight = Quaternion.AngleAxis(90f, Vector3.forward);
startLeft = cam.ViewportToWorldPoint(new Vector3(0, 0.5f, 0.5f));
startRight = cam.ViewportToWorldPoint(new Vector3(1, 0.5f, 0.5f));
startDown = cam.ViewportToWorldPoint(new Vector3(0.5f, 0, 0.5f));
startUp = cam.ViewportToWorldPoint(new Vector3(0.5f, 1, 0.5f));
Arrow fRight = Instantiate<Arrow>(arrow);
Arrow fLeft = Instantiate<Arrow>(arrow);
Arrow fUp = Instantiate<Arrow>(arrow);
Arrow fDown = Instantiate<Arrow>(arrow);
fRight.setDestAndDir(true, KeyCode.D);
fLeft.setDestAndDir(true, KeyCode.A);
fUp.setDestAndDir(true, KeyCode.W);
fDown.setDestAndDir(true, KeyCode.S);
fRight.transform.position = destination;
fLeft.transform.position = destination;
fUp.transform.position = destination;
fDown.transform.position = destination;
fRight.setRotation(rotRight);
fLeft.setRotation(rotLeft);
fUp.setRotation(rotUp);
fDown.setRotation(rotDown);
//cube1.transform.localScale = new Vector3(0.1f, 0.1f, 0.1f);
Arrow arrowRight = Instantiate<Arrow>(arrow);
arrowRight.transform.position = startRight;
arrowRight.setRotation(rotRight);
Arrow arrowDown = Instantiate<Arrow>(arrow);
arrowDown.transform.position = startDown;
arrowDown.setRotation(rotDown);
Arrow arrowLeft = Instantiate<Arrow>(arrow);
arrowLeft.transform.position = startLeft;
arrowLeft.setRotation(rotLeft);
Arrow arrowUp = Instantiate<Arrow>(arrow);
arrowUp.transform.position = startUp;
arrowUp.setRotation(rotUp);
//Arrow arrowDown = Instantiate<Arrow>(arrow);
//arrowDown.transform.position = startDown;
arrowRight.setDestination(destination);
arrowLeft.setDestination(destination);
arrowDown.setDestination(destination);
arrowUp.setDestination(destination);
}
public void setDestination(Vector3 dest)
{
destination = dest;
}
// Update is called once per frame
void Update () {//when evalcuating distance just use sqrmag to save performance
}
}
<file_sep>/Assets/Scripts/LineCreator.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class LineCreator : MonoBehaviour {
public GameObject linePrefab;
public RenderTexture rt;
public Texture2D snowflake;
Line activeLine;
Vector2 bottomLeft, topRight;
bool updateTexture = false;
// Update is called once per frame
void Update () {
if (Input.GetMouseButtonDown(0)) {
GameObject lineGO = Instantiate(linePrefab);
lineGO.transform.SetParent(this.transform);
lineGO.transform.position = Camera.main.transform.position;
lineGO.transform.rotation = Camera.main.transform.rotation;
activeLine = lineGO.GetComponent<Line>();
bottomLeft = topRight = Input.mousePosition;
}
if (Input.GetMouseButtonUp(0)) {
updateTexture = true;
}
if (activeLine != null) {
bottomLeft.x = Mathf.Min(bottomLeft.x, Input.mousePosition.x);
bottomLeft.y = Mathf.Min(bottomLeft.y, Input.mousePosition.y);
topRight.x = Mathf.Max(topRight.x, Input.mousePosition.x);
topRight.y = Mathf.Max(topRight.y, Input.mousePosition.y);
Vector3 mousePos = Camera.main.ScreenToWorldPoint(new Vector3(Input.mousePosition.x, Input.mousePosition.y, Camera.main.nearClipPlane+1.49f));
activeLine.UpdateLine(mousePos);
}
}
private void OnPostRender() {
if (updateTexture) {
snowflake.ReadPixels(new Rect(0,0, rt.width, rt.height),0,0,false);
snowflake.Apply();
snowflake.FloodFillArea(0,0, Color.red);
snowflake.Apply();
activeLine.gameObject.layer += 1; //moved to drawn layer so it's hidden from main camera
activeLine = null;
Debug.Log("BottomLeft Screenspace: " + bottomLeft + ", TopRight: " + topRight);
updateTexture = false;
}
}
}
| a3604cd39bb7eea81103b568a66c075160943a14 | [
"C#"
] | 9 | C# | rhiannanberry/Seven-Dancing-Ghost-Simulators | 3298f481852ed7bd97324f66574f67ddc9ffe13e | 5240078c7c80ed4dd212116bf2827dd3829e0a2f | |
refs/heads/master | <file_sep>'use strict';
//2
let money = window.prompt("Ваш бюджет на месяц?");
let time = prompt("Введите дату в формате YYYY-MM-DD");
//4
let firstExpenses = prompt('Введите обязательную статью расходов в этом месяце');
let firstExpMoney = prompt('Во сколько обойдется?');
let secondExpenses = prompt('Введите обязательную статью расходов в этом месяце');
let secondExpMoney = prompt('Во сколько обойдется?');
//3
let appData = {
budget: money,
timeData: time,
expenses: {
[firstExpenses]: firstExpMoney,
[secondExpenses]: secondExpMoney,
},
optionalExpenses: {
},
income: [],
savings: false,
};
console.log(appData.expenses[firstExpenses]);
console.log(appData.expenses[secondExpenses]);
window.alert("Ваш бюджет: " + appData.budget/30); | e6664866fbaed646be2b2a5be0a25e44f0e464f8 | [
"JavaScript"
] | 1 | JavaScript | albuzi/udemy_react_js_1 | d6430c7ede0abe80bdb88f60d64ee4cb4b2b693d | e6c10a84dc1e56d1dfc79277cd359d613f9a2933 | |
refs/heads/master | <repo_name>ArnalShoorukov/movies2<file_sep>/app/src/main/java/com/example/arnal/movies/AdapterFragment.java
package com.example.arnal.movies;
import android.support.v4.app.Fragment;
import android.content.Context;
import android.support.v4.app.FragmentManager;
import android.support.v4.app.FragmentStatePagerAdapter;
/**
* A simple {@link Fragment} subclass.
*/
public class AdapterFragment extends FragmentStatePagerAdapter {
/** Context of the app */
private Context mContext;
public AdapterFragment(Context context, FragmentManager fm) {
super(fm);
mContext = context;
}
@Override
public android.support.v4.app.Fragment getItem(int position) {
if (position == 0) {
return new PopularFragment();
} else if (position == 1){
return new RatedFragment();
}else
return new FavoriteFragment();
}
@Override
public int getCount() {
return 3;
}
@Override
public CharSequence getPageTitle(int position) {
if(position == 0){
return mContext.getString(R.string.category_popular);
}else if(position == 1){
return mContext.getString(R.string.category_rated);
}else {
return mContext.getString(R.string.category_favorite);
}
}
}
<file_sep>/app/src/main/java/com/example/arnal/movies/MainActivity.java
package com.example.arnal.movies;
import android.support.design.widget.TabLayout;
import android.support.v4.view.ViewPager;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import com.facebook.stetho.Stetho;
public class MainActivity extends AppCompatActivity {
static final String ENDPOINT = "http://api.themoviedb.org/3/";
static final String API_KEY = BuildConfig.MOVIES_TMDB_API_KEY;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Stetho.initializeWithDefaults(this);
// Find the view pager that will allow the user to swipe between fragments
ViewPager viewPager = (ViewPager) findViewById(R.id.viewpager);
AdapterFragment adapter = new AdapterFragment(this, getSupportFragmentManager());
// Set the adapter onto the view pager
viewPager.setAdapter(adapter);
// Find the tab layout that shows the tabs
TabLayout tabLayout = (TabLayout) findViewById(R.id.tabs);
// Connect the tab layout with the view pager. This will
// 1. Update the tab layout when the view pager is swiped
// 2. Update the view pager when a tab is selected
// 3. Set the tab layout's tab names with the view pager's adapter's titles
// by calling onPageTitle()
tabLayout.setupWithViewPager(viewPager);
}
}
<file_sep>/app/src/main/java/com/example/arnal/movies/DetailsFragment.java
package com.example.arnal.movies;
import android.content.ContentValues;
import android.content.Context;
import android.content.UriMatcher;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteOpenHelper;
import android.net.Uri;
import android.os.Bundle;
import android.app.Fragment;
import android.support.design.widget.FloatingActionButton;
import android.support.design.widget.Snackbar;
import android.support.v7.widget.LinearLayoutManager;
import android.support.v7.widget.RecyclerView;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ImageView;
import android.widget.ScrollView;
import android.widget.TextView;
import android.widget.Toast;
import com.example.arnal.movies.adapter.ReviewsAdapter;
import com.example.arnal.movies.adapter.TrailerAdapter;
import com.example.arnal.movies.database.MovieContract;
import com.example.arnal.movies.model.Movie;
import com.example.arnal.movies.model.MoviesAPI;
import com.example.arnal.movies.model.Review;
import com.example.arnal.movies.model.ReviewResult;
import com.example.arnal.movies.model.Trailer;
import com.example.arnal.movies.model.TrailersResult;
import com.squareup.picasso.Picasso;
import java.util.ArrayList;
import retrofit2.Call;
import retrofit2.Callback;
import retrofit2.Response;
import retrofit2.Retrofit;
import retrofit2.converter.gson.GsonConverterFactory;
/**
* A simple {@link Fragment} subclass.
*/
public class DetailsFragment extends Fragment {
private static final String LOG_TAG = DetailsFragment.class.getName();
private static final String ENDPOINT = "http://api.themoviedb.org/3/";
private static final String API_KEY = BuildConfig.MOVIES_TMDB_API_KEY;
static final String POSITION = "position";
static final String FRAGMENT_TYPE = "fragment";
static final String MOVIE_ID = "movieId";
private Movie movie;
MoviesAPI api;
public static ArrayList<Review> reviewList;
public static ArrayList<Trailer> trailerList;
String movieTitle;
int position;
String fragmentType;
String movieId;
Retrofit retrofit;
private ReviewsAdapter adapter;
private TrailerAdapter trailerAdapter;
private Context context;
String id;
public DetailsFragment() {
// Required empty public constructor
}
@Override
public View onCreateView(final LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
final ScrollView scrollView = (ScrollView) inflater.inflate(R.layout.movie_detail_fragment, container, false);
fragmentType = getActivity().getIntent().getStringExtra(FRAGMENT_TYPE);
if (fragmentType != null) {
switch (fragmentType) {
case "PopularFragment":
position = (int) getActivity().getIntent().getExtras().get(POSITION);
movie = PopularFragment.movieList.get(position);
id = movie.getId();
setData(scrollView);
break;
case "RatedFragment":
position = (int) getActivity().getIntent().getExtras().get(POSITION);
movie = RatedFragment.movieList.get(position);
id = movie.getId();
setData(scrollView);
break;
case "FavouriteListFragment":
movieId = getActivity().getIntent().getExtras().getString(MOVIE_ID);
id = movieId;
retrofitCall();
api.getMovies(movieId, API_KEY).enqueue(new Callback<Movie>() {
@Override
public void onResponse(Call<Movie> call, Response<Movie> response) {
movie = response.body();
setData(scrollView);
}
@Override
public void onFailure(Call<Movie> call, Throwable t) {
Toast toast = Toast.makeText(getActivity(), R.string.error, Toast.LENGTH_LONG);
toast.show();
}
});
break;
}
}
FloatingActionButton fab = (FloatingActionButton) scrollView.findViewById(R.id.fab);
fab.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Snackbar.make(view, "Added to Favorite", Snackbar.LENGTH_LONG)
.setAction("Action", null).show();
addFavourite();
}
});
RecyclerView recyclerView = (RecyclerView) scrollView.findViewById(R.id.recyclerView_review);
LinearLayoutManager layoutManager =
new LinearLayoutManager(getActivity(), LinearLayoutManager.VERTICAL, false);
recyclerView.setLayoutManager(layoutManager);
recyclerView.setHasFixedSize(true);
adapter = new ReviewsAdapter(getActivity(), reviewList);
recyclerView.setAdapter(adapter);
RecyclerView recyclerViewTrailer = (RecyclerView) scrollView.findViewById(R.id.recyclerview_trailers);
LinearLayoutManager layoutManager2 =
new LinearLayoutManager(getActivity(), LinearLayoutManager.HORIZONTAL, false);
recyclerViewTrailer.setLayoutManager(layoutManager2);
recyclerViewTrailer.setHasFixedSize(true);
trailerAdapter = new TrailerAdapter(getActivity(), trailerList);
recyclerViewTrailer.setAdapter(trailerAdapter);
getTrailer();
getReviews();
return scrollView;
}
private void getTrailer() {
retrofitCall();
trailerList = new ArrayList<>();
api.getTrailers(id, API_KEY).enqueue(new Callback<TrailersResult>() {
@Override
public void onResponse(Call<TrailersResult> call, Response<TrailersResult> response) {
TrailersResult trailersResult = response.body();
trailerList = (ArrayList<Trailer>) trailersResult.getResults();
trailerAdapter.setTrailerList(trailerList);
}
@Override
public void onFailure(Call<TrailersResult> call, Throwable t) {
Toast toast = Toast.makeText(context, R.string.error, Toast.LENGTH_LONG);
toast.show();
}
});
}
@Override
public void onSaveInstanceState(Bundle outState) {
super.onSaveInstanceState(outState);
}
public void setData(ScrollView scrollView) {
TextView title = (TextView) scrollView.findViewById(R.id.title);
movieTitle = movie.getOriginal_title();
title.setText(movieTitle);
ImageView image = (ImageView) scrollView.findViewById(R.id.iv_details);
Picasso.with(context)
.load("https://image.tmdb.org/t/p/w185/" + movie.getPoster_path())
.into(image);
TextView date = (TextView) scrollView.findViewById(R.id.releaseDate);
String dateMovie = new String(movie.getRelease_date());
date.setText(dateMovie);
TextView overview = (TextView) scrollView.findViewById(R.id.overviewBody);
String overviewMovie = new String(movie.getOverview());
overview.setText(overviewMovie);
Log.i(LOG_TAG, "Show me vote: " + movie.getVote_average());
TextView rate = (TextView) scrollView.findViewById(R.id.vote);
String rating = new String(movie.getVote_average());
rate.setText(rating + "/10");
}
public void retrofitCall() {
retrofit = new Retrofit.Builder()
.baseUrl(ENDPOINT)
.addConverterFactory(GsonConverterFactory.create())
.build();
api = retrofit.create(MoviesAPI.class);
}
public void getReviews() {
retrofitCall();
reviewList = new ArrayList<>();
api.getReview(id, API_KEY).enqueue(new Callback<ReviewResult>() {
@Override
public void onResponse(Call<ReviewResult> call, Response<ReviewResult> response) {
ReviewResult reviewResult = response.body();
reviewList = (ArrayList<Review>) reviewResult.getResults();
adapter.setReviewList(reviewList);
}
@Override
public void onFailure(Call<ReviewResult> call, Throwable t) {
Toast toast = Toast.makeText(getActivity(), R.string.error, Toast.LENGTH_SHORT);
toast.show();
}
});
}
private void addFavourite() {
/*SQLiteOpenHelper dbHelper = new MovieDbHelper(getActivity());
SQLiteDatabase db = dbHelper.getWritableDatabase();
ContentValues contentValues = new ContentValues();
contentValues.put("MOVIE_ID", movie.getId());
contentValues.put("ORIGINAL_TITLE", movie.getOriginal_title());
contentValues.put("POSTER_PATH", movie.getPoster_path());
db.insert("MOVIE", null, contentValues);
db.insertWithOnConflict("MOVIE", null, contentValues, SQLiteDatabase.CONFLICT_IGNORE);*/
//New Content values object
ContentValues contentValues = new ContentValues();
contentValues.put(MovieContract.MovieEntry.COLUMN_TITLE, movie.getOriginal_title());
contentValues.put(MovieContract.MovieEntry.COLUMN_RELEASE_DATE, movie.getRelease_date());
contentValues.put(MovieContract.MovieEntry.COLUMN_VOTE, movie.getVote_average());
contentValues.put(MovieContract.MovieEntry.COLUMN_OVERVIEW, movie.getOverview());
contentValues.put(MovieContract.MovieEntry.COLUMN_POSTER_PATH, movie.getPoster_path());
Uri uri = getActivity().getContentResolver().insert(MovieContract.MovieEntry.CONTENT_URI, contentValues);
/*if(uri != null){
Toast.makeText(getActivity().getApplicationContext().getBaseContext(), uri.toString(),Toast.LENGTH_LONG).show();
}*/
}
}
<file_sep>/app/src/main/java/com/example/arnal/movies/model/MessageEvent.java
package com.example.arnal.movies.model;
public class MessageEvent { //OBJECT IN BUS
public final String fragmentType;
public final int position;
public final int id;
public MessageEvent(int position, String fragmentType, int id) {
this.position = position;
this.fragmentType = fragmentType;
this.id = id;
}
}
<file_sep>/app/src/main/java/com/example/arnal/movies/model/MoviesAPI.java
package com.example.arnal.movies.model;
import retrofit2.Call;
import retrofit2.http.GET;
import retrofit2.http.Path;
import retrofit2.http.Query;
public interface MoviesAPI {
@GET("movie/top_rated")
Call<QueryResult> getFeedTopRated(@Query("api_key") String apiKey);
@GET("movie/popular")
Call<QueryResult> getFeedPopular(@Query("api_key") String apiKey);
@GET("movie/{id}")
Call<Movie> getMovies(@Path("id") String id, @Query("api_key") String apiKey);
@GET("movie/{id}/videos")
Call<TrailersResult> getTrailers(@Path("id") String id, @Query("api_key") String apiKey);
@GET("movie/{id}/reviews")
Call<ReviewResult> getReview(@Path("id") String id, @Query("api_key") String apiKey);
}
| b66b467496d97a5df111a5b386e7f7a1614bc668 | [
"Java"
] | 5 | Java | ArnalShoorukov/movies2 | 8229d576b02e619980530d9e622103ce661a8c4c | 54cedad0efb8bdda471b94e307805d8788e4344b | |
refs/heads/master | <repo_name>boorac/machine-learning<file_sep>/l4/mymlutils.py
from sklearn.metrics import zero_one_loss
import matplotlib.pyplot as plt
def calculate_losses(clf, X_train, X_test, y_train, y_test):
clf.fit(X_train, y_train)
predicted_train = clf.predict(X_train)
predicted_test = clf.predict(X_test)
loss_train = zero_one_loss(y_train, predicted_train)
loss_test = zero_one_loss(y_test, predicted_test)
return loss_train, loss_test
def plot_losses_against_hyperparameter(clfs, hyperparameter_range, hyperparameter_name, X_train, X_test, y_train, y_test, return_optimal=False):
losses_train = []
losses_test = []
figures = []
optimal_loss = [hyperparameter_range[0], float("inf")]
for clf, hyperparameter in zip(clfs, hyperparameter_range):
loss_train, loss_test = calculate_losses(clf, X_train, X_test, y_train, y_test)
losses_train.append(loss_train)
losses_test.append(loss_test)
if return_optimal:
if(optimal_loss[1] > loss_test):
optimal_loss[0] = hyperparameter
optimal_loss[1] = loss_test
fig_loss_test, = plt.plot(hyperparameter_range, losses_test)
fig_loss_train, = plt.plot(hyperparameter_range, losses_train)
plt.legend([fig_loss_test, fig_loss_train], ["test loss", "train_loss"])
plt.ylabel("E")
plt.xlabel(hyperparameter_name)
plt.show()
if return_optimal:
return optimal_loss[0], optimal_loss[1]
| cbb2fdc72fd5a43475a71d165e9fec002a731765 | [
"Python"
] | 1 | Python | boorac/machine-learning | cee1810f07fb5aa9ddce36fedc26acd9a2ce82a8 | 6039e4de31760967328aef73b05d9b792ad3537d | |
refs/heads/master | <repo_name>gustaandrade/MaoNaMassa<file_sep>/Assets/Scripts/GameConstants.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class GameConstants : MonoBehaviour
{
// Tags gerais
public const string PLAYER_TAG = "Player";
public const string LEVEL_TAG = "Level";
public const string DIALOGO_TAG = "Dialogo";
// Valores gerais
public const float PLAYER_SPEED = 2.5F;
// Valores de cena para o Tutorial
public const int ANIM_LIVRO_ON = 4;
public const int ANIM_LIVRO_OFF = 5;
public const int PASSO1_TUTORIAL = 13;
// Pegar água na geladeira
public const int PASSO2_TUTORIAL = 18;
// Levar água para mesa central da neta
public const int PASSO3_TUTORIAL = 21;
// Pegar água da mesa central da vovó
public const int PASSO4_TUTORIAL = 22;
// Levar a água para o fogão
public const int PASSO5_TUTORIAL = 25;
// Pegar gelatina no armário
public const int PASSO6_TUTORIAL = 26;
// Levar gelatina para mesa central da neta
public const int PASSO7_TUTORIAL = 28;
// Buscar água quente no fogão
public const int PASSO8_TUTORIAL = 29;
// Levar água quente para a mesa central da vovó
public const int PASSO9_TUTORIAL = 30;
// Buscar água fria da geladeira
public const int PASSO10_TUTORIAL = 31;
// Levar água fria para a mesa central da neta
public const int PASSO11_TUTORIAL = 33;
// Misturar ingredientes na mesa central
public const int PASSO12_TUTORIAL = 35;
// Levar gelatina para a geladeira
public const int PASSO36_TUTORIAL = 36;
// CursorDaGeladeiraFinal
public const int PASSOFINAL_TUTORIAL = 40;
// Último diálogo do tutorial
public const int VALOR_FORNO = 0;
public const int VALOR_FOGAO = 1;
public const int VALOR_MESAVOVO = 2;
public const int VALOR_ARMARIO = 3;
public const int VALOR_GELADEIRA = 4;
public const int VALOR_PIA = 5;
public const int VALOR_MESANETA = 6;
// Valores de cena para a Receita 2
public const int COUNT_INICIO_RECEITA2 = 9;
// Valores de cena para a Receita 3
public const int COUNT_INICIO_RECEITA3 = 9;
}
<file_sep>/Assets/Scripts/Menu.cs
using UnityEngine;
using System.Collections;
using UnityEngine.SceneManagement;
public class Menu : MonoBehaviour
{
public string Tutorial;
public string Creditos;
public string Receitas;
public void NewGame ()
{
SceneManager.LoadScene (Tutorial);
}
public void Receita ()
{
SceneManager.LoadScene (Receitas);
}
public void CreditsScene ()
{
SceneManager.LoadScene (Creditos);
}
public void Voltar ()
{
SceneManager.LoadScene (1);
}
public void QuitGame ()
{
Application.Quit ();
}
}<file_sep>/Assets/Scripts/MudaPagina.cs
using UnityEngine;
using System.Collections;
using UnityEngine.UI;
using UnityEngine.SceneManagement;
public class MudaPagina : MonoBehaviour
{
public GameObject livro;
public GameObject objLivro;
public GameObject objCreditos;
public GameObject objSair;
private Image imgLivro;
private int flag1 = 0;
private bool flag2 = true;
private bool flag3 = true;
private bool flag4 = true;
public Sprite gelatina;
public Sprite bolinho;
public Sprite chocolate;
void Start ()
{
imgLivro = livro.GetComponent<Image> ();
}
public void TrocarReceita ()
{
flag1++;
if (flag1 == 3)
flag1 = 0;
if (flag1 == 0) {
imgLivro.sprite = gelatina;
} else if (flag1 == 1) {
imgLivro.sprite = bolinho;
} else if (flag1 == 2) {
imgLivro.sprite = chocolate;
}
}
public void TrocarCanvasReceita ()
{
if (!flag2) {
objLivro.SetActive (false);
flag2 = true;
} else {
objLivro.SetActive (true);
flag2 = false;
}
}
public void TrocarCanvasInfo ()
{
if (!flag3) {
objCreditos.SetActive (false);
flag3 = true;
} else {
objCreditos.SetActive (true);
flag3 = false;
}
}
public void TrocarCanvasSair ()
{
if (!flag4) {
objSair.SetActive (false);
flag4 = true;
} else {
objSair.SetActive (true);
flag4 = false;
}
}
public void LoadReceita ()
{
if (flag1 == 0) {
SceneManager.LoadScene (2);
} else if (flag1 == 1) {
SceneManager.LoadScene (4);
} else if (flag1 == 2) {
SceneManager.LoadScene (5);
}
}
}<file_sep>/Assets/Scripts/Pause.cs
using UnityEngine;
using System.Collections;
using UnityEngine.SceneManagement;
public class Pause : MonoBehaviour {
public string Menu;
public AudioSource soundManager;
public GameObject thePauseScreen;
public GameObject toqueCollider;
void Start () {
soundManager = GameObject.Find ("SoundManager").GetComponent<AudioSource>();
}
void Update () {
if(Input.GetButtonDown("Pause"))
{
if(Time.timeScale == 0f)
{
ResumeGame();
} else {
PauseGame();
}
}
}
public void PauseGame()
{
Time.timeScale = 0;
thePauseScreen.SetActive(true);
toqueCollider.SetActive (false);
soundManager.Pause();
}
public void ResumeGame()
{
thePauseScreen.SetActive(false);
Time.timeScale = 1f;
toqueCollider.SetActive (true);
soundManager.Play ();
}
public void QuitToMainMenu()
{
Time.timeScale = 1f;
SceneManager.LoadScene(Menu);
}
}<file_sep>/Assets/Scripts/DialogControl.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using UnityEngine.SceneManagement;
public class DialogControl : MonoBehaviour
{
public GameObject dialogos;
private Transform log;
private Text[] vetorDialogos;
private int numDialogos;
public GameObject canvasLivro;
private Animator livro;
public Button next;
public int count;
public GameObject aguafria1;
public GameObject aguafria2;
public GameObject aguafria3;
public GameObject aguafria4;
public GameObject aguaquente1;
public GameObject aguaquente2;
public GameObject aguavermelha;
public GameObject bowl;
public GameObject pogelatina1;
public GameObject pogelatina2;
public GameObject gelatinafinal;
public GameObject btnMix;
public Button btnNext;
/**************** RECEITA 2 *****************/
public TutorialControl receita;
public GameObject canvasDialogo;
public AudioSource etapaSom;
public AudioClip etapaSon;
private bool soundPlayed = false;
void Start ()
{
etapaSom = GetComponent<AudioSource> ();
log = dialogos.GetComponent<Transform> ();
numDialogos = log.childCount;
vetorDialogos = new Text[numDialogos];
for (int i = 0; i < numDialogos; i++) {
vetorDialogos [i] = log.GetChild (i).gameObject.GetComponent<Text> ();
}
livro = canvasLivro.GetComponent<Animator> ();
}
void Update ()
{
ChangeDialog ();
}
public void ChangeDialog ()
{
/**************** RECEITA 1 *****************/
if (SceneManager.GetActiveScene ().buildIndex == 2) {
if (count == GameConstants.ANIM_LIVRO_ON) {
livro.Play ("anim_fadein_livro");
}
if (count == GameConstants.ANIM_LIVRO_OFF) {
livro.Play ("anim_fadeout_livro");
}
if (count == GameConstants.PASSO1_TUTORIAL
|| count == GameConstants.PASSO2_TUTORIAL
|| count == GameConstants.PASSO3_TUTORIAL
|| count == GameConstants.PASSO4_TUTORIAL
|| count == GameConstants.PASSO5_TUTORIAL
|| count == GameConstants.PASSO6_TUTORIAL
|| count == GameConstants.PASSO7_TUTORIAL
|| count == GameConstants.PASSO8_TUTORIAL
|| count == GameConstants.PASSO9_TUTORIAL
|| count == GameConstants.PASSO10_TUTORIAL
|| count == GameConstants.PASSO11_TUTORIAL
|| count == GameConstants.PASSO12_TUTORIAL) {
next.interactable = false;
}
if (count == GameConstants.PASSO1_TUTORIAL + 1) {
aguafria1.SetActive (true);
if (!soundPlayed) {
etapaSom.PlayOneShot (etapaSon);
soundPlayed = true;
}
} else if (count == GameConstants.PASSO2_TUTORIAL + 1) {
aguafria1.SetActive (false);
aguafria2.SetActive (true);
TocarSom ();
} else if (count == GameConstants.PASSO3_TUTORIAL + 1) {
aguafria2.SetActive (false);
aguafria4.SetActive (true);
TocarSom ();
} else if (count == GameConstants.PASSO4_TUTORIAL + 1) {
aguafria4.SetActive (false);
aguafria3.SetActive (true);
TocarSom ();
} else if (count == 24) {
aguafria3.SetActive (false);
aguaquente1.SetActive (true);
TocarSom ();
} else if (count == GameConstants.PASSO5_TUTORIAL + 1) {
aguafria3.SetActive (false);
pogelatina1.SetActive (true);
TocarSom ();
} else if (count == GameConstants.PASSO6_TUTORIAL + 1) {
pogelatina1.SetActive (false);
pogelatina2.SetActive (true);
TocarSom ();
} else if (count == GameConstants.PASSO8_TUTORIAL + 1) {
aguaquente1.SetActive (false);
aguaquente2.SetActive (true);
TocarSom ();
} else if (count == GameConstants.PASSO9_TUTORIAL + 1) {
aguafria1.SetActive (true);
TocarSom ();
} else if (count == GameConstants.PASSO10_TUTORIAL + 1) {
aguafria1.SetActive (false);
aguafria2.SetActive (true);
TocarSom ();
} else if (count == GameConstants.PASSO11_TUTORIAL) {
btnMix.SetActive (true);
} else if (count == GameConstants.PASSO11_TUTORIAL + 1) {
btnMix.SetActive (false);
btnNext.interactable = true;
aguafria2.SetActive (false);
aguaquente2.SetActive (false);
pogelatina2.SetActive (false);
bowl.SetActive (false);
aguavermelha.SetActive (true);
} else if (count == GameConstants.PASSO12_TUTORIAL + 1) {
aguavermelha.SetActive (false);
gelatinafinal.SetActive (true);
TocarSom ();
} else if (count == GameConstants.PASSOFINAL_TUTORIAL) {
SceneManager.LoadScene (3);
}
}
/**************** RECEITA 2 *****************/
else if (SceneManager.GetActiveScene ().buildIndex == 4) {
if (count == GameConstants.ANIM_LIVRO_ON) {
livro.Play ("anim_fadein_livro");
}
if (count == GameConstants.ANIM_LIVRO_OFF) {
livro.Play ("anim_fadeout_livro");
}
if (count == GameConstants.COUNT_INICIO_RECEITA2) {
receita.SetAllBoxesTrue ();
canvasDialogo.GetComponent<Canvas> ().enabled = false;
}
}
/**************** RECEITA 3 *****************/
else if (SceneManager.GetActiveScene ().buildIndex == 5) {
if (count == GameConstants.ANIM_LIVRO_ON) {
livro.Play ("anim_fadein_livro");
}
if (count == GameConstants.ANIM_LIVRO_OFF) {
livro.Play ("anim_fadeout_livro");
}
if (count == GameConstants.COUNT_INICIO_RECEITA3) {
receita.SetAllBoxesTrue ();
canvasDialogo.GetComponent<Canvas> ().enabled = false;
}
}
}
public void SetCount ()
{
count++;
vetorDialogos [count - 1].GetComponent<Animator> ().Play ("anim_fadeout_text");
vetorDialogos [count - 1].enabled = false;
vetorDialogos [count].GetComponent<Animator> ().Play ("anim_fadein_text");
vetorDialogos [count].enabled = true;
Debug.Log (count);
soundPlayed = false;
}
public int GetCount ()
{
return count;
}
public void TocarSom ()
{
if (!soundPlayed) {
etapaSom.PlayOneShot (etapaSon);
soundPlayed = true;
}
}
}
<file_sep>/Assets/Scripts/Tap.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using TouchScript.Gestures;
public class Tap : MonoBehaviour
{
private TapGesture gesture;
public PlayerController player;
// Use this for initialization
void Awake ()
{
gesture = GetComponent<TapGesture> ();
}
// Update is called once per frame
void TapHandler (object sender, System.EventArgs e)
{
player.EscolherDestino (gameObject.name);
}
public void OnEnable ()
{
gesture.Tapped += TapHandler;
}
public void OnDisable ()
{
gesture.Tapped -= TapHandler;
}
}
<file_sep>/Assets/Scripts/SplashScreen.cs
using UnityEngine;
using System.Collections;
using UnityEngine.SceneManagement;
public class SplashScreen : MonoBehaviour {
public float timer = 4f;
public string Menu;
void Start()
{
StartCoroutine ("LoadMenu");
}
IEnumerator LoadMenu()
{
yield return new WaitForSeconds (timer);
SceneManager.LoadScene(Menu);
}
}
<file_sep>/Assets/Scripts/PlayerController.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using UnityEngine.SceneManagement;
public class PlayerController : MonoBehaviour
{
/**************** RECEITA 1 *****************/
public Transform posicaoFogao;
public Transform posicaoForno;
public Transform posicaoMesaVovo;
public Transform posicaoArmario;
public Transform posicaoGeladeira;
public Transform posicaoPia;
public Transform posicaoMesaNeta;
private Transform destino1;
private Transform destino2;
public Transform origDestino1;
public Transform origDestino2;
public GameObject player1;
public GameObject player2;
private bool flagMover1 = false;
private bool flagMover2 = false;
public TutorialControl tutorial;
private Transform[] numeroPosicoes;
private Animator animVo;
private Animator animNeta;
private BoxCollider2D boxVo;
private BoxCollider2D boxNeta;
#region DECLARAÇÕES RECEITA 2
/**************** RECEITA 2 *****************/
public GameObject canvasArmario;
public GameObject canvasGeladeira;
public GameObject btnAcucar;
public GameObject btnFarinha;
public GameObject btnOleo;
public GameObject btnOvo;
public GameObject btnLeite;
public GameObject btnMix;
private bool flagEtapa1 = false;
private bool flagEtapa2 = false;
private bool flagEtapa3 = false;
public GameObject ovos;
public GameObject farinha;
public GameObject leite;
public GameObject oleo1;
public GameObject oleo2;
public GameObject massa1;
public GameObject massa2;
public GameObject massa3;
public GameObject bolinho1;
public GameObject bolinho2;
public GameObject bolinho3;
public GameObject panela1;
public GameObject panela2;
public GameObject acucar;
public GameObject bolinhofinal;
public GameObject btnMix2;
public GameObject relogio1;
#endregion
#region DECLARAÇÕES RECEITA 3
/**************** RECEITA 3 *****************/
public GameObject r3_canvasArmario;
public GameObject r3_canvasGeladeira;
public GameObject r3_btnAcucar1;
public GameObject r3_btnAcucar2;
public GameObject r3_btnFarinha;
public GameObject r3_btnOvo;
public GameObject r3_btnLeite;
public GameObject r3_btnChocolate1;
public GameObject r3_btnChocolate2;
public GameObject r3_btnManteiga1;
public GameObject r3_btnManteiga2;
public GameObject r3_btnFermento;
public GameObject r3_btnCreme;
public GameObject r3_btnMix;
public GameObject r3_btnMix2;
public GameObject r3_ovos;
public GameObject r3_farinha;
public GameObject r3_leite;
public GameObject r3_acucar1;
public GameObject r3_acucar2;
public GameObject r3_acucar3;
public GameObject r3_fermento;
public GameObject r3_manteiga1;
public GameObject r3_manteiga2;
public GameObject r3_manteiga3;
public GameObject r3_chocolate1;
public GameObject r3_chocolate2;
public GameObject r3_chocolate3;
public GameObject r3_massaBolo1;
public GameObject r3_massaBolo2;
public GameObject r3_bolo1;
public GameObject r3_bolo2;
public GameObject r3_creme1;
public GameObject r3_creme2;
public GameObject r3_panela1;
public GameObject r3_panela2;
public GameObject r3_calda;
public GameObject r3_boloFinal;
public GameObject relogio2;
public GameObject relogio3;
private bool flagEtapa1_R3 = false;
private bool flagEtapa2_R3 = false;
private bool flagEtapa3_R3 = false;
private bool flagEtapa4_R3 = false;
private bool flagEtapa5_R3 = false;
private bool flagEtapa6_R3 = false;
#endregion
#region DECLARAÇÕES CANVASCHEERS PARA R2 E R3
public GameObject canvasCheers;
public GameObject txtr2_1;
public GameObject txtr2_2;
public GameObject txtr2_3;
public GameObject btnFim1;
public GameObject txtr3_1;
public GameObject txtr3_2;
public GameObject txtr3_3;
public GameObject btnFim2;
#endregion
void Start ()
{
Input.multiTouchEnabled = true;
destino1 = origDestino1;
destino2 = origDestino2;
numeroPosicoes = new Transform[7];
numeroPosicoes [0] = posicaoForno;
numeroPosicoes [1] = posicaoFogao;
numeroPosicoes [2] = posicaoMesaVovo;
numeroPosicoes [3] = posicaoArmario;
numeroPosicoes [4] = posicaoGeladeira;
numeroPosicoes [5] = posicaoPia;
numeroPosicoes [6] = posicaoMesaNeta;
}
void Update ()
{
MoverPersonagem ();
}
public void EscolherDestino (string name)
{
if (name == "Forno") {
destino1 = posicaoForno;
if (SceneManager.GetActiveScene ().buildIndex == 5) {
LevarForno_R3 ();
}
AnimeVovo (1);
flagMover1 = true;
} else if (name == "Fogao") {
destino1 = posicaoFogao;
if (SceneManager.GetActiveScene ().buildIndex == 4) {
LevarFogao ();
} else if (SceneManager.GetActiveScene ().buildIndex == 5) {
LevarFogao_R3 ();
}
AnimeVovo (2);
flagMover1 = true;
} else if (name == "MesaVovo") {
destino1 = posicaoMesaVovo;
if (SceneManager.GetActiveScene ().buildIndex == 4) {
PegarMesa ();
} else if (SceneManager.GetActiveScene ().buildIndex == 5) {
PegarMesa_R3 ();
}
AnimeVovo (3);
flagMover1 = true;
}
if (name == "Armario") {
destino2 = posicaoArmario;
if (SceneManager.GetActiveScene ().buildIndex == 4) {
canvasArmario.GetComponent<Canvas> ().enabled = true;
canvasGeladeira.GetComponent<Canvas> ().enabled = false;
} else if (SceneManager.GetActiveScene ().buildIndex == 5) {
r3_canvasArmario.GetComponent<Canvas> ().enabled = true;
r3_canvasGeladeira.GetComponent<Canvas> ().enabled = false;
}
AnimeNeta (3);
flagMover2 = true;
} else if (name == "Geladeira") {
destino2 = posicaoGeladeira;
if (SceneManager.GetActiveScene ().buildIndex == 4) {
canvasArmario.GetComponent<Canvas> ().enabled = false;
canvasGeladeira.GetComponent<Canvas> ().enabled = true;
} else if (SceneManager.GetActiveScene ().buildIndex == 5) {
r3_canvasArmario.GetComponent<Canvas> ().enabled = false;
r3_canvasGeladeira.GetComponent<Canvas> ().enabled = true;
}
AnimeNeta (1);
flagMover2 = true;
} else if (name == "Pia") {
destino2 = posicaoPia;
if (SceneManager.GetActiveScene ().buildIndex == 4) {
canvasArmario.GetComponent<Canvas> ().enabled = false;
canvasGeladeira.GetComponent<Canvas> ().enabled = false;
} else if (SceneManager.GetActiveScene ().buildIndex == 5) {
r3_canvasArmario.GetComponent<Canvas> ().enabled = false;
r3_canvasGeladeira.GetComponent<Canvas> ().enabled = false;
}
AnimeNeta (3);
flagMover2 = true;
} else if (name == "MesaNeta") {
destino2 = posicaoMesaNeta;
if (SceneManager.GetActiveScene ().buildIndex == 4) {
canvasArmario.GetComponent<Canvas> ().enabled = false;
canvasGeladeira.GetComponent<Canvas> ().enabled = false;
ColocarNaMesa ();
} else if (SceneManager.GetActiveScene ().buildIndex == 5) {
r3_canvasArmario.GetComponent<Canvas> ().enabled = false;
r3_canvasGeladeira.GetComponent<Canvas> ().enabled = false;
ColocarNaMesa_R3 ();
}
AnimeNeta (2);
flagMover2 = true;
}
}
public void MoverPersonagem ()
{
if (flagMover1) {
player1.transform.position = Vector3.MoveTowards (player1.transform.position, destino1.position, GameConstants.PLAYER_SPEED * Time.deltaTime);
if (Vector3.Distance (player1.transform.position, destino1.position) < 0.1) {
AnimeVovo (5);
tutorial.SetFlagMover1 ();
flagMover1 = false;
}
}
if (flagMover2) {
player2.transform.position = Vector3.MoveTowards (player2.transform.position, destino2.position, GameConstants.PLAYER_SPEED * Time.deltaTime);
if (Vector3.Distance (player2.transform.position, destino2.position) < 0.1) {
AnimeNeta (5);
tutorial.SetFlagMover2 ();
flagMover2 = false;
}
}
}
public void AnimeVovo (int i)
{
if (i == 1) {
player1.GetComponent<Animator> ().SetBool ("isWest", false);
player1.GetComponent<Animator> ().SetBool ("isEast", false);
player1.GetComponent<Animator> ().SetBool ("isSouth", false);
player1.GetComponent<Animator> ().SetBool ("isNorth", true);
}
if (i == 2) {
player1.GetComponent<Animator> ().SetBool ("isNorth", false);
player1.GetComponent<Animator> ().SetBool ("isEast", false);
player1.GetComponent<Animator> ().SetBool ("isSouth", false);
player1.GetComponent<Animator> ().SetBool ("isWest", true);
}
if (i == 3) {
player1.GetComponent<Animator> ().SetBool ("isNorth", false);
player1.GetComponent<Animator> ().SetBool ("isWest", false);
player1.GetComponent<Animator> ().SetBool ("isSouth", false);
player1.GetComponent<Animator> ().SetBool ("isEast", true);
}
if (i == 4) {
player1.GetComponent<Animator> ().SetBool ("isNorth", false);
player1.GetComponent<Animator> ().SetBool ("isWest", false);
player1.GetComponent<Animator> ().SetBool ("isEast", false);
player1.GetComponent<Animator> ().SetBool ("isSouth", true);
}
if (i == 5) {
player1.GetComponent<Animator> ().SetBool ("isNorth", false);
player1.GetComponent<Animator> ().SetBool ("isWest", false);
player1.GetComponent<Animator> ().SetBool ("isEast", false);
player1.GetComponent<Animator> ().SetBool ("isSouth", false);
}
}
public void AnimeNeta (int i)
{
if (i == 1) {
player2.GetComponent<Animator> ().SetBool ("isWest", false);
player2.GetComponent<Animator> ().SetBool ("isEast", false);
player2.GetComponent<Animator> ().SetBool ("isSouth", false);
player2.GetComponent<Animator> ().SetBool ("isNorth", true);
}
if (i == 2) {
player2.GetComponent<Animator> ().SetBool ("isNorth", false);
player2.GetComponent<Animator> ().SetBool ("isEast", false);
player2.GetComponent<Animator> ().SetBool ("isSouth", false);
player2.GetComponent<Animator> ().SetBool ("isWest", true);
}
if (i == 3) {
player2.GetComponent<Animator> ().SetBool ("isNorth", false);
player2.GetComponent<Animator> ().SetBool ("isWest", false);
player2.GetComponent<Animator> ().SetBool ("isSouth", false);
player2.GetComponent<Animator> ().SetBool ("isEast", true);
}
if (i == 4) {
player2.GetComponent<Animator> ().SetBool ("isNorth", false);
player2.GetComponent<Animator> ().SetBool ("isWest", false);
player2.GetComponent<Animator> ().SetBool ("isEast", false);
player2.GetComponent<Animator> ().SetBool ("isSouth", true);
}
if (i == 5) {
player2.GetComponent<Animator> ().SetBool ("isNorth", false);
player2.GetComponent<Animator> ().SetBool ("isWest", false);
player2.GetComponent<Animator> ().SetBool ("isEast", false);
player2.GetComponent<Animator> ().SetBool ("isSouth", false);
}
}
#region FUNÇÕES RECEITA 2
/**************** RECEITA 2 *****************/
public void EscolherItem1 ()
{
btnAcucar.SetActive (false);
canvasArmario.GetComponent<Canvas> ().enabled = false;
}
public void EscolherItem2 ()
{
btnFarinha.SetActive (false);
canvasArmario.GetComponent<Canvas> ().enabled = false;
}
public void EscolherItem3 ()
{
btnOleo.SetActive (false);
canvasArmario.GetComponent<Canvas> ().enabled = false;
}
public void EscolherItem4 ()
{
btnOvo.SetActive (false);
canvasArmario.GetComponent<Canvas> ().enabled = false;
}
public void EscolherItem5 ()
{
btnLeite.SetActive (false);
canvasGeladeira.GetComponent<Canvas> ().enabled = false;
}
public void ColocarNaMesa ()
{
if (!flagEtapa1) {
if (btnOvo.activeInHierarchy == false) {
ovos.SetActive (true);
}
if (btnFarinha.activeInHierarchy == false) {
farinha.SetActive (true);
}
if (btnLeite.activeInHierarchy == false) {
leite.SetActive (true);
}
if (btnOleo.activeInHierarchy == false) {
oleo1.SetActive (true);
}
if (btnAcucar.activeInHierarchy == false) {
acucar.SetActive (true);
}
if (btnAcucar.activeInHierarchy == false && btnFarinha.activeInHierarchy == false &&
btnOleo.activeInHierarchy == false && btnOvo.activeInHierarchy == false &&
btnLeite.activeInHierarchy == false) {
btnMix.SetActive (true);
StartCoroutine (CheersR2 (1));
}
}
}
public void MisturarIngredientes ()
{
StartCoroutine (Rotina ());
}
IEnumerator Rotina ()
{
flagEtapa1 = true;
btnMix.SetActive (false);
ovos.SetActive (false);
farinha.SetActive (false);
leite.SetActive (false);
massa1.SetActive (true);
yield return new WaitForSecondsRealtime (3);
massa1.SetActive (false);
massa2.SetActive (true);
}
public void PegarMesa ()
{
if (massa2.activeInHierarchy == true) {
oleo1.SetActive (false);
massa2.SetActive (false);
flagEtapa2 = true;
}
if (flagEtapa3) {
panela1.SetActive (false);
bolinho2.SetActive (false);
panela2.SetActive (true);
bolinho3.SetActive (true);
btnMix2.SetActive (true);
flagEtapa3 = false;
}
}
public void LevarFogao ()
{
if (flagEtapa2) {
tutorial.SetBoxFalse (1);
oleo2.SetActive (true);
massa3.SetActive (true);
StartCoroutine (FritarBolinho ());
}
}
IEnumerator FritarBolinho ()
{
yield return new WaitForSecondsRealtime (3);
relogio1.SetActive (true);
oleo2.SetActive (false);
massa3.SetActive (false);
//panela1.SetActive (true);
bolinho1.SetActive (true);
StartCoroutine (CheersR2 (2));
yield return new WaitForSecondsRealtime (3);
relogio1.SetActive (false);
bolinho1.SetActive (false);
bolinho2.SetActive (true);
flagEtapa2 = false;
flagEtapa3 = true;
}
public void TerminarReceita ()
{
acucar.SetActive (false);
panela2.SetActive (false);
bolinho3.SetActive (false);
btnMix2.SetActive (false);
bolinhofinal.SetActive (true);
StartCoroutine (CheersR2 (3));
}
IEnumerator CheersR2 (int num)
{
canvasCheers.SetActive (true);
if (num == 1) {
txtr2_1.SetActive (true);
yield return new WaitForSecondsRealtime (3);
canvasCheers.SetActive (false);
} else if (num == 2) {
txtr2_1.SetActive (false);
txtr2_2.SetActive (true);
yield return new WaitForSecondsRealtime (3);
canvasCheers.SetActive (false);
} else if (num == 3) {
txtr2_2.SetActive (false);
txtr2_3.SetActive (true);
btnFim1.SetActive (true);
}
}
#endregion
/**************** RECEITA 3 *****************/
#region FUNÇÕES RECEITA 3
public void EscolherItem1_R3 ()
{
r3_btnChocolate1.SetActive (false);
r3_canvasArmario.GetComponent<Canvas> ().enabled = false;
}
public void EscolherItem2_R3 ()
{
r3_btnFarinha.SetActive (false);
r3_canvasArmario.GetComponent<Canvas> ().enabled = false;
}
public void EscolherItem3_R3 ()
{
r3_btnOvo.SetActive (false);
r3_canvasArmario.GetComponent<Canvas> ().enabled = false;
}
public void EscolherItem4_R3 ()
{
r3_btnFermento.SetActive (false);
r3_canvasArmario.GetComponent<Canvas> ().enabled = false;
}
public void EscolherItem5_R3 ()
{
r3_btnAcucar1.SetActive (false);
r3_canvasArmario.GetComponent<Canvas> ().enabled = false;
}
public void EscolherItem6_R3 ()
{
r3_btnManteiga1.SetActive (false);
r3_canvasGeladeira.GetComponent<Canvas> ().enabled = false;
}
public void EscolherItem7_R3 ()
{
r3_btnLeite.SetActive (false);
r3_canvasGeladeira.GetComponent<Canvas> ().enabled = false;
}
public void EscolherItem8_R3 ()
{
r3_btnChocolate2.SetActive (false);
r3_canvasArmario.GetComponent<Canvas> ().enabled = false;
}
public void EscolherItem9_R3 ()
{
r3_btnCreme.SetActive (false);
r3_canvasArmario.GetComponent<Canvas> ().enabled = false;
}
public void EscolherItem10_R3 ()
{
r3_btnAcucar2.SetActive (false);
r3_canvasArmario.GetComponent<Canvas> ().enabled = false;
}
public void EscolherItem11_R3 ()
{
r3_btnManteiga2.SetActive (false);
r3_canvasGeladeira.GetComponent<Canvas> ().enabled = false;
}
public void ColocarNaMesa_R3 ()
{
if (!flagEtapa1_R3) {
if (r3_btnChocolate1.activeInHierarchy == false) {
r3_chocolate1.SetActive (true);
}
if (r3_btnFarinha.activeInHierarchy == false) {
r3_farinha.SetActive (true);
}
if (r3_btnOvo.activeInHierarchy == false) {
r3_ovos.SetActive (true);
}
if (r3_btnFermento.activeInHierarchy == false) {
r3_fermento.SetActive (true);
}
if (r3_btnAcucar1.activeInHierarchy == false) {
r3_acucar1.SetActive (true);
}
if (r3_btnManteiga1.activeInHierarchy == false) {
r3_manteiga1.SetActive (true);
}
if (r3_btnLeite.activeInHierarchy == false) {
r3_leite.SetActive (true);
}
if (r3_btnChocolate1.activeInHierarchy == false && r3_btnFarinha.activeInHierarchy == false &&
r3_btnOvo.activeInHierarchy == false && r3_btnFermento.activeInHierarchy == false &&
r3_btnAcucar1.activeInHierarchy == false && r3_btnManteiga1.activeInHierarchy == false &&
r3_btnLeite.activeInHierarchy == false) {
r3_btnMix.SetActive (true);
}
}
if (r3_bolo2.activeInHierarchy == true) {
if (r3_btnChocolate2.activeInHierarchy == false) {
r3_chocolate2.SetActive (true);
}
if (r3_btnAcucar2.activeInHierarchy == false) {
r3_acucar2.SetActive (true);
}
if (r3_btnManteiga2.activeInHierarchy == false) {
r3_manteiga2.SetActive (true);
}
if (r3_btnCreme.activeInHierarchy == false) {
r3_creme1.SetActive (true);
}
if (r3_btnChocolate2.activeInHierarchy == false && r3_btnAcucar2.activeInHierarchy == false &&
r3_btnManteiga2.activeInHierarchy == false && r3_btnCreme.activeInHierarchy == false) {
flagEtapa5_R3 = true;
}
}
}
public void MisturarIngredientes_R3 ()
{
flagEtapa1_R3 = true;
r3_btnMix.SetActive (false);
r3_ovos.SetActive (false);
r3_chocolate1.SetActive (false);
r3_manteiga1.SetActive (false);
r3_farinha.SetActive (false);
r3_acucar1.SetActive (false);
r3_leite.SetActive (false);
r3_fermento.SetActive (false);
r3_massaBolo1.SetActive (true);
StartCoroutine (CheersR3 (1));
}
public void PegarMesa_R3 ()
{
if (r3_massaBolo1.activeInHierarchy == true) {
r3_massaBolo1.SetActive (false);
flagEtapa2_R3 = true;
}
if (flagEtapa3_R3) {
r3_bolo1.SetActive (false);
r3_bolo2.SetActive (true);
StartCoroutine (CheersR3 (2));
flagEtapa3_R3 = false;
flagEtapa4_R3 = true;
LigarElementosCalda ();
}
if (r3_bolo2.activeInHierarchy == true && r3_chocolate2.activeInHierarchy == true &&
r3_acucar2.activeInHierarchy == true && r3_manteiga2.activeInHierarchy == true &&
r3_creme1.activeInHierarchy == true) {
r3_chocolate2.SetActive (false);
r3_acucar2.SetActive (false);
r3_manteiga2.SetActive (false);
r3_creme1.SetActive (false);
flagEtapa5_R3 = true;
}
if (flagEtapa6_R3) {
r3_panela2.SetActive (false);
r3_calda.SetActive (true);
r3_btnMix2.SetActive (true);
tutorial.SetBoxFalse (2);
tutorial.SetBoxFalse (5);
}
}
public void LevarForno_R3 ()
{
if (flagEtapa2_R3) {
r3_massaBolo2.SetActive (true);
StartCoroutine (AssarBolo ());
}
}
public IEnumerator AssarBolo ()
{
tutorial.SetBoxFalse (0);
yield return new WaitForSecondsRealtime (3);
relogio2.SetActive (true);
r3_massaBolo2.SetActive (false);
yield return new WaitForSecondsRealtime (3);
relogio2.SetActive (false);
r3_bolo1.SetActive (true);
flagEtapa2_R3 = false;
flagEtapa3_R3 = true;
}
public void LigarElementosCalda ()
{
if (flagEtapa4_R3) {
r3_btnChocolate2.SetActive (true);
r3_btnAcucar2.SetActive (true);
r3_btnManteiga2.SetActive (true);
r3_btnCreme.SetActive (true);
}
}
public void LevarFogao_R3 ()
{
if (flagEtapa5_R3) {
r3_chocolate3.SetActive (true);
r3_acucar3.SetActive (true);
r3_manteiga3.SetActive (true);
r3_creme2.SetActive (true);
StartCoroutine (FazerCalda ());
}
}
IEnumerator FazerCalda ()
{
tutorial.SetBoxFalse (1);
yield return new WaitForSecondsRealtime (3);
relogio3.SetActive (true);
r3_chocolate3.SetActive (false);
r3_acucar3.SetActive (false);
r3_manteiga3.SetActive (false);
r3_creme2.SetActive (false);
yield return new WaitForSecondsRealtime (3);
relogio3.SetActive (false);
r3_panela1.SetActive (false);
r3_panela2.SetActive (true);
flagEtapa6_R3 = true;
}
public void TerminarReceita_R3 ()
{
r3_bolo2.SetActive (false);
r3_calda.SetActive (false);
r3_btnMix2.SetActive (false);
r3_boloFinal.SetActive (true);
StartCoroutine (CheersR3 (3));
}
IEnumerator CheersR3 (int num)
{
canvasCheers.SetActive (true);
if (num == 1) {
txtr3_1.SetActive (true);
yield return new WaitForSecondsRealtime (3);
canvasCheers.SetActive (false);
} else if (num == 2) {
txtr3_1.SetActive (false);
txtr3_2.SetActive (true);
yield return new WaitForSecondsRealtime (3);
canvasCheers.SetActive (false);
} else if (num == 3) {
txtr3_2.SetActive (false);
txtr3_3.SetActive (true);
btnFim2.SetActive (true);
}
}
#endregion
}
<file_sep>/Assets/Scripts/SceneChanger.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
public class SceneChanger : MonoBehaviour
{
public void EncerrarReceita ()
{
SceneManager.LoadScene (3);
}
void Update ()
{
if (Input.GetKeyDown (KeyCode.F2)) {
SceneManager.LoadScene (0);
}
if (Input.GetKeyDown (KeyCode.F3)) {
SceneManager.LoadScene (1);
}
if (Input.GetKeyDown (KeyCode.F4)) {
SceneManager.LoadScene (2);
}
if (Input.GetKeyDown (KeyCode.F5)) {
SceneManager.LoadScene (3);
}
if (Input.GetKeyDown (KeyCode.F6)) {
SceneManager.LoadScene (4);
}
if (Input.GetKeyDown (KeyCode.F7)) {
SceneManager.LoadScene (5);
}
}
}
<file_sep>/Assets/Scripts/SomButton.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class SomButton : MonoBehaviour
{
private AudioSource music;
public Sprite btnOn;
public Sprite btnOff;
private Image btn;
void Awake ()
{
music = GetComponent<AudioSource> ();
btn = GameObject.Find ("Som").GetComponent<Image> ();
}
public void ChangeSound ()
{
if (music.mute) {
music.mute = false;
btn.sprite = btnOn;
} else {
music.mute = true;
btn.sprite = btnOff;
}
}
}<file_sep>/Assets/Scripts/TrocarCanvas.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
public class TrocarCanvas : MonoBehaviour
{
public GameObject canvasReceita;
public void LigarCanvasReceita ()
{
canvasReceita.SetActive (true);
}
public void DesligarCanvasReceita ()
{
canvasReceita.SetActive (false);
}
public void LoadReceita1 ()
{
SceneManager.LoadScene (2);
}
public void LoadReceita2 ()
{
SceneManager.LoadScene (4);
}
public void LoadReceita3 ()
{
SceneManager.LoadScene (5);
}
}
<file_sep>/README.md
# MaoNaMassa
Repositório para o jogo Mão na Massa, desenvolvido em Unity 2D, para a plataforma Android.
<file_sep>/Assets/Scripts/FadeControl.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class FadeControl : MonoBehaviour
{
public Canvas intro;
public Canvas inicio;
public GameObject panel;
public void Fade ()
{
panel.SetActive (true);
}
public void Off ()
{
intro.gameObject.SetActive (false);
inicio.gameObject.SetActive (true);
}
public void Begin ()
{
inicio.gameObject.SetActive (false);
}
}
<file_sep>/Assets/Scripts/TutorialControl.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using UnityEngine.SceneManagement;
public class TutorialControl : MonoBehaviour
{
public DialogControl dc;
public Button btn;
public PlayerController pc;
public BoxCollider2D forno;
public BoxCollider2D fogao;
public BoxCollider2D mesaVovo;
public BoxCollider2D armario;
public BoxCollider2D geladeira;
public BoxCollider2D pia;
public BoxCollider2D mesaNeta;
public bool flagMover1;
public bool flagMover2;
private int proximoBox1;
private int proximoBox2;
public GameObject btnMix;
public SpriteRenderer cursorFogao;
public SpriteRenderer cursorGeladeira;
public SpriteRenderer cursorArmario;
public SpriteRenderer cursorMesaVo;
public SpriteRenderer cursorMesaNeta;
private bool flagBoxes = false;
void Update ()
{
if (SceneManager.GetActiveScene ().buildIndex == 2) {
if (dc.GetCount () == GameConstants.PASSO1_TUTORIAL) {
geladeira.enabled = true;
cursorGeladeira.enabled = true;
DefinirProximoDestino2 (GameConstants.VALOR_MESANETA);
TestFlags (geladeira);
} else if (dc.GetCount () == GameConstants.PASSO2_TUTORIAL) {
cursorGeladeira.enabled = false;
mesaNeta.enabled = true;
cursorMesaNeta.enabled = true;
DefinirProximoDestino2 (GameConstants.VALOR_ARMARIO);
TestFlags (mesaNeta);
} else if (dc.GetCount () == GameConstants.PASSO3_TUTORIAL) {
cursorMesaNeta.enabled = false;
mesaVovo.enabled = true;
cursorMesaVo.enabled = true;
DefinirProximoDestino1 (GameConstants.VALOR_FOGAO);
TestFlags (mesaVovo);
} else if (dc.GetCount () == GameConstants.PASSO4_TUTORIAL) {
cursorMesaVo.enabled = false;
fogao.enabled = true;
cursorFogao.enabled = true;
DefinirProximoDestino1 (GameConstants.VALOR_FOGAO);
TestFlags (fogao);
} else if (dc.GetCount () == GameConstants.PASSO5_TUTORIAL) {
cursorFogao.enabled = false;
armario.enabled = true;
cursorArmario.enabled = true;
DefinirProximoDestino2 (GameConstants.VALOR_MESANETA);
TestFlags (armario);
} else if (dc.GetCount () == GameConstants.PASSO6_TUTORIAL) {
cursorArmario.enabled = false;
mesaNeta.enabled = true;
cursorMesaNeta.enabled = true;
DefinirProximoDestino2 (GameConstants.VALOR_GELADEIRA);
TestFlags (mesaNeta);
} else if (dc.GetCount () == GameConstants.PASSO7_TUTORIAL) {
cursorMesaNeta.enabled = false;
fogao.enabled = true;
cursorFogao.enabled = true;
DefinirProximoDestino1 (GameConstants.VALOR_MESAVOVO);
TestFlags (fogao);
} else if (dc.GetCount () == GameConstants.PASSO8_TUTORIAL) {
cursorFogao.enabled = false;
mesaVovo.enabled = true;
cursorMesaVo.enabled = true;
DefinirProximoDestino1 (GameConstants.VALOR_MESAVOVO);
TestFlags (mesaVovo);
} else if (dc.GetCount () == GameConstants.PASSO9_TUTORIAL) {
cursorMesaVo.enabled = false;
geladeira.enabled = true;
cursorGeladeira.enabled = true;
DefinirProximoDestino2 (GameConstants.VALOR_MESANETA);
TestFlags (geladeira);
} else if (dc.GetCount () == GameConstants.PASSO10_TUTORIAL) {
cursorGeladeira.enabled = false;
mesaNeta.enabled = true;
cursorMesaNeta.enabled = true;
DefinirProximoDestino2 (GameConstants.VALOR_MESANETA);
TestFlags (mesaNeta);
} else if (dc.GetCount () == GameConstants.PASSO12_TUTORIAL) {
cursorMesaNeta.enabled = false;
geladeira.enabled = true;
cursorGeladeira.enabled = true;
TestFlags (geladeira);
} else if (dc.GetCount () == GameConstants.PASSO36_TUTORIAL) {
cursorGeladeira.enabled = false;
}
}
}
public void TestFlags (BoxCollider2D box)
{
if (SceneManager.GetActiveScene ().buildIndex == 2) {
if (flagMover1) {
dc.SetCount ();
btn.interactable = true;
flagMover1 = false;
DeactivateObject (box);
} else if (flagMover2) {
dc.SetCount ();
btn.interactable = true;
flagMover2 = false;
DeactivateObject (box);
}
}
}
public void SetFlagMover1 ()
{
flagMover1 = true;
}
public void SetFlagMover2 ()
{
flagMover2 = true;
}
public void DeactivateObject (BoxCollider2D box)
{
box.enabled = false;
}
public void DefinirProximoDestino1 (int num1)
{
proximoBox1 = num1;
}
public void DefinirProximoDestino2 (int num2)
{
proximoBox2 = num2;
}
public int MandarPosicaoBox1 ()
{
return proximoBox1;
}
public int MandarPosicaoBox2 ()
{
return proximoBox2;
}
public void SetAllBoxesTrue ()
{
if (!flagBoxes) {
forno.enabled = true;
fogao.enabled = true;
mesaVovo.enabled = true;
armario.enabled = true;
geladeira.enabled = true;
mesaNeta.enabled = true;
flagBoxes = true;
}
}
public void SetBoxFalse (int num)
{
if (num == 0) {
forno.enabled = false;
} else if (num == 1) {
fogao.enabled = false;
} else if (num == 2) {
mesaVovo.enabled = false;
} else if (num == 3) {
armario.enabled = false;
} else if (num == 4) {
geladeira.enabled = false;
} else if (num == 5) {
mesaNeta.enabled = false;
}
}
} | 4aceb31df9e5dc999bad9db6fcb02783a3d70c71 | [
"Markdown",
"C#"
] | 14 | C# | gustaandrade/MaoNaMassa | 90c39f576708300f2e91b53484fc8ae204b09695 | c9ccb634f3e01b81f1e269db69ef698caa5278d9 | |
refs/heads/master | <file_sep>'''
Created on 2019年10月17日
@author: yxr
'''
import requests
from orca.debug import println
"""
requests库的7个主要方法
request() 构造一个请求,支撑一下各方法的基础方法
get() 获取HTML网页的主要方法,对应于HTTP的get方法
head()获取HTML网页头信息,对应于HTTP的HEAD
post() post请求
put() put请求
patch() 向HTML网页提交局部修改请求,对应于HTTP的patch请求
delete() delete请求
req.encoding 如果header中不存在charset,则认为编码为ISO-88591-1
req.apparent_encoding 根据网页内容分析出的编码方式
"""
def test_01():
req = requests.get("http://www.baidu.com")
code = req.status_code #响应码,200 SUCCESS,404 FILE
print("响应码-->",code)
print("当前编码:"+req.encoding)
req.encoding = "utf-8" #content encoding
print("修改后编码:"+req.encoding)
respMsg = req.text #HTTP response message
print("响应信息-->",respMsg)
print("\n")
print( req.content) #response binary message
"""
理解requests 库的异常
requests.ConnectionError :网络链接错误异常,如DNS查询失败,拒绝连接等
requests.HTTPError :HTTP错误
requests.URLRuired : URL缺失异常
requests.TooManyRedirects 超过最大重定向次数,产生从定向异常
requests.ConnectTimeout :连接远程服务超时异常
request.Timeout :请求URL超时,产生超时异常
requests.raise_for_status() 如果不是200,产生异常requests.HTTPError
"""
# 爬取网页的通用代码方法
def test_02(url):
try:
r = requests.get(url,timeout=30)
r.raise_for_status() #如果不是200,产生异常requests.HTTPError
r.encoding = r.apparent_encoding
return r.text
except:
return "Exception"
#-----------------------------------------------------------------------------
"""
HHTP协议对资源的操作
1)get : 请求获取URL位置的资源
2)head :请求回去URL位置资源的响应消息报告,即获得该资源的头部信息
3)post :请求向URL位置的资源后,附加新的数据
4)put : 请求向URL位置存储一个资源,覆盖原URL位置的资源
5)patch : 请求局部更新URL位置资源,即改变该处资源的部分内容
6)delete : 请求删除URL位置存储的资源
"""
"""
{
'Cache-Control': 'private, no-cache, no-store, proxy-revalidate, no-transform',
'Connection': 'keep-alive', 'Content-Encoding': 'gzip',
'Content-Type': 'text/html',
'Date': 'Sat, 19 Oct 2019 12:27:13 GMT',
'Last-Modified': 'Mon, 13 Jun 2016 02:50:26 GMT',
'Pragma': 'no-cache',
'Server': 'bfe/192.168.127.12'}
"""
def test_head():
r =requests.head("https://www.baidu.com/")
heads = r.headers
print(r.headers)
text = r.text
print(text) #空的
"""
{
"args": {},
"data": "",
"files": {},
"form": {
"age": "15",
"name": "xiaobai"
},
"headers": {
"Accept": "*/*",
"Accept-Encoding": "gzip, deflate",
"Content-Length": "19",
"Content-Type": "application/x-www-form-urlencoded",
"Host": "httpbin.org",
"User-Agent": "python-requests/2.22.0"
},
"json": null,
"origin": "172.16.17.32, 172.16.17.32",
"url": "https://httpbin.org/post"
}
"""
def test_post():
payload = {'name':'xiaobai','age':'15'}
r = requests.post("http://httpbin.org/post", data = payload)
print(r.text)
"""
{
"args": {},
"data": "",
"files": {},
"form": {
"age": "15",
"name": "xiaobai"
},
"headers": {
"Accept": "*/*",
"Accept-Encoding": "gzip, deflate",
"Content-Length": "19",
"Content-Type": "application/x-www-form-urlencoded",
"Host": "httpbin.org",
"User-Agent": "python-requests/2.22.0"
},
"json": null,
"origin": "172.16.17.32, 172.16.17.32",
"url": "https://httpbin.org/put"
}
"""
def test_put():
payload = {'name':'xiaobai','age':'15'}
r = requests.put("http://httpbin.org/put", data = payload)
print(r.text)
#-----------------------------------------------------------------------------
"""
requests.request(method,url,**kwarge)
method:请求方式,对应get/put/post/put/patch/delete/options等7种,
url : 获取页面的url链接
**kwargs:控制访问的参数,共13个
1)params:字典或 字节序列,作为参数增加到url中
kv = {'name':'xiaobai','age':'15'}
url = 'http://python123.io/ws'
r = requests.request('GET',url,params = kv)
print(r.url)
2)data:字典,字节序列或文件对象,作为request的内容
kv = {'name':'xiaobai','age':'15'}
url = 'http://python123.io/ws'
r = requests.request('GET',url,data = kv)
print(r.url)
3)json: json格式数据,做为request的内容
kv = {'name':'xiaobai','age':'15'}
url = 'http://python123.io/ws'
r = requests.request('GET',url,json = kv)
print(r.url)
4)headers:字典,http定制头
hd = { "User-Agent": "Chrome/10"}
url = 'http://python123.io/ws'
r = requests.request('GET',url,headers = hd)
5)cookies:字典或者CookieJar,requests中的cookies
6)auth: 元组,支持http认证功能
7)files:字典类型,传输文件
fs = {'file':open('data.xls','rb')}
url = 'http://python123.io/ws'
r = request.request('post',url,files =fs)
8)timeout:设置超时时间,秒为单位
url = 'http://python123.io/ws'
r = request.request('post',url,timeout = 10)
9)proxies:字典类型,设定访问代理服务器,可以增加登入认证
pxs = {
'http':'http://user:[email protected]:1234'
'http':'https://10.10.10.1:4321'
}
r = requests.request('GET','http://www.baidu.com',proxies = pxs)
10)allow_redirects:True/False ,默认为True,重定向开关
11)stream : True/False,默认为True,获取内容立即下载开关
12)verify: True/False,默认为True,认证SSL证书开关
13)cert:本地SSL证书路径
"""
def test_params():
kv = {'name':'xiaobai','age':'15'}
url = 'http://python123.io/ws'
r = requests.request('GET',url,params = kv)
print(r.url)#https://python123.io/ws?name=xiaobai&age=15
#-----------------------------------------------------------------------------
"""
网络爬虫的尺寸:
1)爬取网页,玩转网页:小规模,数据量小,爬取速度不敏感,requests库
2)爬取系列网站:中规模,数据规模较大,爬取速度敏感,scrapy库
3)爬取全网:大规模,搜索引擎,爬取速度关键,需要定制开发
网络爬虫的限制:
1)来源审查:判断User-Agent进行限制
检查来访HTTP协议头的User-Agent域,只响应浏览器或友好的爬虫的访问
2)发布公告:Robots协议
告知所有爬虫网站的爬去策略,要求爬虫遵循
ROBOTS协议
1)Robots Exclusion Standard :网络爬虫排除标准
2)作用:告知所有爬虫网站的爬去策略,要求爬虫遵循
3)方式:在网站根目录下的rebots.txt文件
robots使用:
网络爬虫:自动或人工识别robots.txt,再进行内容爬取
约束性:robots协议是建议但非约束性,网络爬虫可以不遵守,但存在法律风险
"""
if __name__ == "__main__" :
test_params()
# test_put()
# test_post()
# test_head()
# test_01()
# url = "http://www.baidu.com"
# print(test_02(url))
<file_sep>'''
Created on 2019年10月19日
@author: yxr
'''
import requests
def test_jd():
url = "https://item.jd.com/100006635632.html"
try:
r = requests.get(url)
r.raise_for_status()
r.encoding = r.apparent_encoding
print(r.text)
except:
print("爬去失败")
def test_amazon():
url = "https://www.amazon.cn/dp/B07Y9C684P"
try:
kv = {'user-agent':'Mozilla/5.0 (X11; Ubuntu; Linux x86_64; rv:69.0) Gecko/20100101 Firefox/69.0'}
r = requests.get(url,headers = kv)
r.raise_for_status()
r.encoding = r.apparent_encoding
print(r.text)
except:
print("fail")
def test_baidu():
kv = {'wd':'python'}
try:
r = requests.get("http://www.baidu.com/s",params= kv)
print(r.request.url)
r.raise_for_status()
print(len(r.text))
except:
print("fail")
if __name__ == "__main__" :
test_baidu()
| e29b495f6e7700922d2494067c63a3fc7988eea1 | [
"Python"
] | 2 | Python | yinxianren/python_study | 149608f6c73ba043bc0bcd940e8a9dddac283e3a | 6851bdd189099489cb36d010e1b174a67dcf0993 | |
refs/heads/master | <repo_name>sargentogato/SQL-Queries<file_sep>/README.md
# SQL Queries
### Nivel I
### Nivel II
### Nivel III
<file_sep>/BBDDmysql.sql
/* Ejercicio 1 */
SELECT COUNT(`FlightNum`) AS Total FROM `flights`
/* 2 ejercicio */
SELECT `Origin`, AVG(`ArrDelay`), AVG(`DepDelay`)
FROM `flights`
GROUP BY `Origin`
/* 3 ejercicio */
SELECT `Origin`, `colYear`, `colMonth`, AVG(`ArrDelay`)
AS `prom_arribades` FROM `flights`
GROUP BY `Origin`, `colYear`, `colMonth`
/* 4 ejercicio */
SELECT City, colYear, colMonth, AVG(ArrDelay) AS prom_arribades
FROM flights
INNER JOIN usairports
ON flights.Origin=usairports.IATA
GROUP BY
`City`, `colYear`, `colMonth`
/* 5 ejercicio */
SELECT `UniqueCarrier`, `colYear`, `colMonth`, SUM(cancelled) AS total_cancelled
FROM `flights`
WHERE Cancelled > 0
GROUP BY UniqueCarrier, colYear, colMonth
ORDER BY COUNT(*) DESC
/* 6 ejercicio */
SELECT TailNum, SUM(Distance) AS totalDistance
FROM flights
WHERE NOT TailNum='NULL'
GROUP BY TailNum
ORDER BY totalDistance DESC LIMIT 10
/* 7 ejercicio */
SELECT UniqueCarrier, AVG(ArrDelay)
FROM flights
GROUP BY UniqueCarrier
HAVING AVG(ArrDelay) > 10
ORDER BY AVG(ArrDelay) DESC | 1f084c559d7fc73f2da20cb4983edd8d922b4973 | [
"Markdown",
"SQL"
] | 2 | Markdown | sargentogato/SQL-Queries | 8c81a787c748f9931d87a83628d1a8bbd3002204 | e4d732fac570ef738990faf60cdf3b95be965edd | |
refs/heads/master | <repo_name>andmmtel/telegram_bot<file_sep>/tgram.py
from telegram.ext import Updater, CommandHandler, MessageHandler, Filters
from apikey import API_KEY
qa={"привет": "И тебе привет!",
"как дела": "Лучше всех",
"пока": "Увидимся"}
def get_answer(question):
return qa.get(str(question).lower().strip(' ?!,:'),'Не понимаю')
def start(bot, update):
print('Вызван /start')
bot.sendMessage(update.message.chat_id, text='Привет, человек! Я тупой бот, который умеет повторять то, что ты скажешь! Давай общаться!')
def whoami(bot, update):
tuser=update.message.from_user
print('Вызван /whoami ('+tuser.first_name+' '+tuser.last_name+')')
bot.sendMessage(update.message.chat_id, text='Ты - '+tuser.first_name+' '+tuser.last_name)
def talk_to_me(bot, update):
print('Пришло сообщение: ' + update.message.text)
print('От: ' + update.message.from_user.username+' '+update.message.from_user.first_name)
answer=get_answer(update.message.text)
bot.sendMessage(update.message.chat_id, answer)
print('Послано сообщение:'+ answer)
#answer=input('Какой ответ? ')
#bot.sendMessage(update.message.chat_id, answer)
#print('Послано сообщение:'+ answer)
#bot.sendMessage(update.message.chat_id, update.message.text)
#print('Послано сообщение:'+ update.message.text)
def run_bot():
updater = Updater(API_KEY)
dp = updater.dispatcher
dp.add_handler(CommandHandler("start", start))
dp.add_handler(CommandHandler("whoami", whoami))
dp.add_handler(MessageHandler([Filters.text], talk_to_me))
updater.start_polling()
updater.idle()
if __name__ == '__main__':
run_bot() | a41aee5f40a140ba6d033432bf97968e3c27a405 | [
"Python"
] | 1 | Python | andmmtel/telegram_bot | 8b358a8af0e1a5308d3cf88240c24f39dbb59374 | 9c1d714a25ddb461bf1ed04cdd35ccbfa5b82e0c | |
refs/heads/master | <file_sep><?php
echo 'Ohoho PHP! *kotz*';
?><file_sep>GIT WDD 414
===========
Testrepository für GIT Demonstration im SAE Kurs WDD414.
Beispiel Überschrift 2
----------------------
Auch ein bisschen *Markdown* im Unterricht. Hat noch **niemandem** geschadet.
```php
<?php
echo 'Ja, genau! haha. not.';
?>
```
Weitere Beispiele sehen Sie in der beigelegten index.php. Für weitere Fragen wenden Sie sich an Ihren Arzt oder Apotheker.
```js
console.log('winwin');
```<file_sep>console.log("Ein JS Beispiel…"); | f80513a8e3691121b9eb86ab65bf412433b71e39 | [
"Markdown",
"JavaScript",
"PHP"
] | 3 | PHP | originell/git-wdd414 | 62a5f7a57274ffbf6f33acb63ec056cd70f5ba7a | 02c9a7c92c03be09f5fad308389559a1c417374d | |
refs/heads/main | <repo_name>kavithaponugupati05/conditional<file_sep>/app/views.py
from django.shortcuts import render
# Create your views here.
def hai(request):
d={'a':220,'b':530,'c':100}
return render(request,'hai.html',d)
| bab55716ec668962f159e403ed7bca91a035e21f | [
"Python"
] | 1 | Python | kavithaponugupati05/conditional | 2e45dbbed6d96ba4ed1b0d53c1daa50e7a8ae164 | 3c8326b725310c5ccca1039e84d4a08ff50d4117 | |
refs/heads/master | <repo_name>cran/FinAna<file_sep>/man/plotsm.Rd
\name{plotsm}
\alias{plotsm}
\title{Plot scatter smooth plots for a data.frame}
\usage{
plotsm(x,dependent,c,l)
}
\arguments{
\item{x}{:a dataframe}
\item{dependent}{:the dependent variable}
\item{c}{:is there dummy variable in the data.frame; c = 0 when there is none; c = 1 when there is}
\item{l}{: number of labeling starts at (default = 1)}
}
\description{
Plotting scatter smooth plots for a data.frame, with name, number and labels.
}
\examples{
# plotsm(JPM-ratios,"price"",0,20)
}
<file_sep>/R/kur.R
###############################################################################################
##### kurtosis
###############################################################################################
#' @name kur
#' @aliases kur
#' @title Calculating kurtosis for numeric data
#' @description Kurtosis
#' @usage kur(x)
#' @param x :a numeric variable
#' @examples #kur(return) for skewness of variable return
kur <- function(x){
x <- na.omit(x)
me <- mean(x,na.rm = T)
med <- median(x, na.rm = T)
std <- sd(x,na.rm = T)
kurtosis <- mean((x - me)^4)/(mean((x-me)^2)^2)
return(kurtosis)
}
<file_sep>/man/get.price.google.Rd
\name{get.price.google}
\alias{get.price.google}
\title{Download financial data from google finance}
\usage{
get.price.google(tkr, bg = "2001-01-01",ed = "today")
}
\arguments{
\item{tkr}{:company ticker, e.g. "BABA","AMZN"}
\item{bg}{:beginning date, e.g."2000-02-29"}
\item{ed}{:ending date, e.g. "today", "2016-11-10"}
}
\description{
Download stock prices for one company or a list of companies from google finance. And furthur application of rate of return function and beta function in the package for more analysis.
}
\examples{
#get.price.google("GOOG")
#get.price.google("GOOG", bg = "2001-01-01",ed = "today")
# the two above are the same
#
# tkr <- c("AAPL", "IBM","YHOO")
# pricelist <- get.price.google(tkr, bg = "2001-01-01",ed = "today")
# aapl <- pricelist[1] # convert to single data.frame
# ibm <- pricelist[2] # convert to single data.frame
# yhoo <- pricelist[3] # convert to single data.frame
}
<file_sep>/R/get.price.google.R
###############################################################################################
##### Download financial data from google
###############################################################################################
get.price.google <- function (tkr, bg = "2001-01-01", ed = "today"){
n <- length(tkr)
pricelist <- list()
bgmth <- month.abb[as.numeric(substring(bg, 6,7))]
bgday <- substring(bg,9,10)
bgyear <- substring(bg,1,4)
if (ed != "today") {
edmth <- month.abb[as.numeric(substring(ed, 6,7))]
edday <- substring(ed,9,10)
edyear <- substring(ed,1,4)
}
else {
ed <- as.character(Sys.Date())
edmth <- month.abb[as.numeric(substring(ed, 6,7))]
edday <- substring(ed,9,10)
edyear <- substring(ed,1,4)
}
for (i in 1:n) {
p <- read.csv(paste0("https://www.google.com/finance/historical?q=", tkr[i], "&output=csv", "&startdate=", bgmth, "+", bgday, "+", bgyear, "&enddate=", edmth, "+", edday, "+", edyear))
names(p) <- c("date", "open", "high", "low", "close", "volume")
p$obs <- 1 : length(p$open)
p <- p[sort(p$obs, decreasing = T),]
p <- p[,1:6]
p$obs <- 1 : length(p$open)
}
pricelist[[tkr[i]]] = p
return(pricelist)
}
<file_sep>/R/plotfhs.R
##################################################################################################
### Ploting Function
##################################################################################################
## function to create histogram and scatter plots for data.frame:
## a = 0 for both
## a = 1 for histogram
## a = 2 for scatter plots
## dependent is the column name of the dependent variable
## c = 0 when there is no dummy varibale in the data.frame
## c = 1 when there is dummy varibale in the data.frame
#' @name ploths
#' @aliases ploths
#' @title Plot histograms and scatter plots for a data.frame
#' @description Plotting histograms or scatter plots of your choice for a data.frame. Also the function will name the graphs and number them.The purpose of the function is to save time when plotting graphs for a regression analysis or other usage. The function can plot, name and number the graphs at one step.
#' @usage ploths(x,a,dependent,c,l)
#' @param x :a dataframe
#' @param a :the type of graph you want; a = 1 for histograms; a = 2 for scatter plots; a = 0 for both
#' @param dependent :the dependent variable for scatterplots
#' @param c :is there dummy variable in the dataframe; c = 0 when there is none; c = 1 when there is
#' @param l : number of labeling starts at (default = 1)
#' @examples #ploths(sp500,0,"price",0,20)
ploths <- function(x,a,dependent,c,l = 1){
yl <- dependent
dependent <- x[,dependent]
typeofvar <- sapply(x,class)
ha <- typeofvar[typeofvar == "numeric" & !names(typeofvar) == dependent]
if(c == 1){
dumornot <- typeofvar[typeofvar == "integer"]
dummyvar <- x[,c(names(dumornot))]
dummyvar <- dummyvar[!unique(dummyvar) == 0 & !unique(dummyvar) == 1]
ha <- c(ha,dummyvar)
}
x <- x[,c(names(ha))]
var <- names(x)
n <- length(var)
if (a == 1){
hist(dependent, main = paste("Fig.", paste(l, paste("Histogram of",dependent))), xlab = yl)
for(i in 1:n){
hist(x[,i], main = paste("Fig.", paste(i+l, paste("Histogram of",var[i]))), xlab = var[i])
}
}else if(a == 2){
for(i in 1:n){
plot(x[,i], dependent, main = paste("Fig.", paste(i, paste("Scatter Plot of",var[i]))), xlab = var[i], ylab = yl)
}
}else{
hist(dependent, main = paste("Fig.", paste(l, paste("Histogram of",dependent))), xlab = yl)
for(i in 1:n){
hist(x[,i], main = paste("Fig.", paste(i+l, paste("Histogram of",var[i]))), xlab = var[i])}
for(i in 1:n){
plot(x[,i], dependent, main = paste("Fig.", paste(i+n+l, paste("Scatter Plot of",var[i]))), xlab = var[i], ylab = yl)
}
}
}
<file_sep>/man/getmode.Rd
\name{get.mode}
\alias{get.mode}
\title{Calculating mode for numeric data}
\usage{
get.mode(x)
}
\arguments{
\item{x}{:a numeric variable(vector)}
}
\description{
Calculating mode for numeric data
}
\examples{
# get.mode(return)
}
<file_sep>/R/annu.pv.R
##################################################################################################
### Annuity Present Value
##################################################################################################
#' @name annu.pv
#' @aliases annu.pv
#' @title Calculate present value of annuity
#' @description Calculate present value of an ordinary annuity or an annuity due.
#' @usage annu.pv(pmt,i,n,type = 0)
#' @param pmt :the equal amount of payment of each period
#' @param i :interest rate accoding to the period
#' @param n :number of periods
#' @param type :type = 0 for ordinary annuity, type = 1 for annuity due
#' @examples #annu.pv(100,0.0248,10,0)
annu.pv <- function(pmt,i,n,type = 0){
if(type == 1){
pv <- pmt*((1-((1+i)^-n))/i)*(1+i)
}else{
pv <- pmt*((1-((1+i)^-n))/i)
}
return(pv)
}
<file_sep>/R/betaf.R
###############################################################################################
##### Beta for companies
###############################################################################################
#' @name betaf
#' @aliases betaf
#' @title Calculating beta for a company or a select of companies
#' @description Calculating beta using common method or linear regression(OLS)
#' @usage betaf(x,y,method)
#' @param x :a vector or a data.frame of rate of return of companies
#' @param y :name of the independent variable
#' @param method :method of calculation; method = 1 for a common expression of beta(see detail); method = 2 using linear regression to estimate the beta
#' @examples #betaf(appl,sp500)
betaf <- function(x,y,method){
dependent <- x[,y]
typeofvar <- sapply(x,class)
ha <- typeofvar[typeofvar == "numeric" & !names(typeofvar) == y]
x <- x[,c(names(ha))]
if(method == 1){
n <- dim(x)[2]
varr <- var(dependent,na.rm = T)
betare <- as.data.frame(matrix(nrow = n, ncol = 2))
betare[,1] <- names(x)
for (i in 1:n){
covdf <- as.data.frame(cbind(x[,i],dependent))
covdf <- as.data.frame(na.omit(covdf))
covv <- cov(covdf[1], covdf[2], use = "na.or.complete")
betare[i,2] <- covv/varr
}
}else{
n <- dim(x)[2]
betare <- as.data.frame(matrix(nrow = n, ncol = 2))
betare[,1] <- names(x)
for(i in 1:n){
rrco <- x[,i]
rdf <- as.data.frame(cbind(rrco, dependent))
lmFit <- lm(dependent~rrco, rdf, na.omit = na.action)
betare[i,2] <- lmFit$coefficients[2]
}
}
return(betare)
}
<file_sep>/R/rr.R
###############################################################################################
##### Calculating rate of return of a vector
###############################################################################################
#' @name rr
#' @aliases rr
#' @title Calculating rate of return of a vector
#' @description Calculating the rate of return of a vector for further analysis, including calculating beta of companies, plotting to see the trend of the stock for technical analysis
#' @usage rr(x,n)
#' @param x :a vector of company prices
#' @param n : number of lags
#' @examples #rr(aapl,1)
rr <- function(x, n){
ratereturn <- c(as.numeric("NA"),(lag(as.ts(x), k = n)/as.ts(x))-1)
return(ratereturn)
}
<file_sep>/R/desc.R
###############################################################################################
##### Descriptive statistics
###############################################################################################
#' @name desc
#' @aliases desc
#' @title Descriptice statistics of a data.frame
#' @description Calculating the descriptive statistics of a data.frame and exporting in a data.frame
#' @usage desc(x,n)
#' @param x :a data.frame
#' @param n :number of decimal points
#' @examples #desc(sp1500,3) for descriptive statistics of sp1500
desc <- function(x,n){
typeofvar <- sapply(x,class)
ha <- names(typeofvar[typeofvar == "numeric" | typeofvar == "integer"])
x <- x[,c(ha)]
des <- as.data.frame(matrix(nrow = dim(x)[2], ncol = 11))
names(des) <- c("name","obs","max","min","mean","median","mode","var","std","skew","kurt")
des[,1] <- names(x)
des[,2] <- round(apply(x,2, length), n)
des[,3] <- round(apply(x,2, max, na.rm = T), n)
des[,4] <- round(apply(x,2, min, na.rm = T), n)
des[,5] <- round(apply(x,2,mean, na.rm = T), n)
des[,6] <- round(apply(x,2,median, na.rm = T), n)
des[,7] <- round(apply(x,2,get.mode), n)
des[,8] <- round(apply(x,2,var, na.rm = T), n)
des[,9] <- round(apply(x,2,sd, na.rm = T), n)
des[,10] <- round(apply(x,2,sk), n)
des[,11] <- round(apply(x,2,kur), n)
return(des)
}
<file_sep>/man/get.price.yahoo.Rd
\name{get.price.yahoo}
\alias{get.price.yahoo}
\title{Download financial data from Yahoo finance}
\usage{
get.price.yahoo(tkr, bg = "first",ed = "today", f = "d")
}
\arguments{
\item{tkr}{:company ticker, e.g. "BABA","AMZN"}
\item{bg}{:beginning date, e.g. "first","2000-02-29"}
\item{ed}{:ending date, e.g. "today", "2016-11-10"}
\item{f}{:frequency, e.g. "d" for daily,"w" for weekly,"m" for monthly}
}
\description{
Download stock prices for one company or a list of companies from Yahoo finance. The function can download daily, weekly and monthly data. And furthur application of rate of return function and beta function in the package for more analysis.
}
\examples{
#get.price.yahoo("GOOG")
#get.price.yahoo("GOOG", bg = "first",ed = "today", f = "d")
# the two above are the same
#
# tkr <- c("AAPL", "IBM","YHOO")
# pricelist <- get.price.yahoo(tkr, bg = "first",ed = "today", f = "m")
# aapl <- pricelist[1] # convert to single data.frame
# ibm <- pricelist[2] # convert to single data.frame
# yhoo <- pricelist[3] # convert to single data.frame
}
<file_sep>/R/plotsm.R
##################################################################################################
### Ploting Function scatter smooth
##################################################################################################
plotsm <- function(x,dependent,c,l = 1){
x <- na.omit(x)
yl <- dependent
dependent <- x[,dependent]
typeofvar <- sapply(x,class)
ha <- typeofvar[typeofvar == "numeric"]
if(c == 1){
dumornot <- typeofvar[typeofvar == "integer"]
dummyvar <- x[,c(names(dumornot))]
dummyvar <- as.data.frame(dummyvar[!unique(dummyvar) == 0 & !unique(dummyvar) == 1])
if(dim(dummyvar)[1] > 0){
ha <- c(dumornot,ha)}
}
x <- x[,c(names(ha))]
var <- names(x)
n <- length(var)
x <- as.data.frame(na.omit(x))
for(i in 1:n){
scatter.smooth(x[,i],dependent, main = paste("Fig.", paste(i+l-1, paste("Scatter Plot of",var[i]))), xlab = var[i],ylab = yl)
}
}
<file_sep>/R/corm.R
###############################################################################################
##### Correlation Matrix
###############################################################################################
#' @name corm
#' @aliases corm
#' @title Correlation matrix and correlation ranking of a data.frame
#' @description Calculating the descriptive statistics of a data.frame and exporting in a data.frame
#' @usage corm(x,n)
#' @param x :a data.frame
#' @param n :number of decimal points
#' @examples #corm(sp1500,3) for correlation matrix of sp1500
corm <- function(x,n){
typeofvar <- sapply(x,class)
ha <- names(typeofvar[typeofvar == "numeric" | typeofvar == "integer" | typeofvar == "double"])
x <- x[,c(ha)]
cormatirx <-data.frame(round(cor(x, use = "na.or.complete"), n))
corrank <- data.frame(sort(cor(x,use = "na.or.complete")[,1],decreasing = T))
return(cormatirx)
return(corrank)
}
<file_sep>/R/get.price.yahoo.R
###############################################################################################
##### Download financial data from yahoo
###############################################################################################
get.price.yahoo <- function (tkr, bg = "first", ed = "today", f = "d"){
num <- length(tkr)
pricelist <- list()
if (bg == "first" & ed == "today") {
for (i in 1:num) {
p <- read.csv(paste0("http://ichart.finance.yahoo.com/table.csv?s=", tkr[i]))
p <- p[order(p$Date),]
names(p) <- c("date", "open", "high", "low", "close", "volume", "adjusted")
p$obs <- c(1:length(p[,1]))
pricelist[[tkr[i]]] <- p
}
}else{
bgmth <- as.character(as.numeric(substring(bg,6,7))-1)
bgday <- substring(bg,9,10)
bgyear <- substring(bg,1,4)
if (ed != "today") {
edmth <- as.character(as.numeric(substring(ed,6,7))-1)
edday <- substring(ed,9,10)
edyear <- substring(ed,1,4)
}else{
ed <- as.character(substring(Sys.time(),1,10))
edmth <- substring(ed,6,7)
edday <- substring(ed,9,10)
edyear <- substring(ed,1,4)
}
for (i in 1:num) {
p <- read.csv(paste0("http://ichart.finance.yahoo.com/table.csv?s=", tkr[i], "&a=", bgmth, "&b=", bgday, "&c=", bgyear, "&d=", edmth, "&e=", edday, "&f=", edyear, "&g=", f))
p <- p[order(p$Date),]
names(p) <- c("date", "open", "high", "low", "close", "volume", "adjusted")
p$obs <- c(1:length(p[,1]))
pricelist[[tkr[i]]] <- p
}
}
return(pricelist)
}
<file_sep>/R/ploth.R
##################################################################################################
### Ploting Function
##################################################################################################
## function to create histogram and scatter plots for data.frame:
## c = 0 when there is no dummy varibale in the data.frame
## c = 1 when there is dummy varibale in the data.frame
#' @name ploth
#' @aliases ploth
#' @title Plot histograms for a data.frame
#' @description Plotting histograms for a data.frame. Also the function will name the graphs and number the graphs.
#' @usage ploth(x,c,l)
#' @param x :a dataframe
#' @param c :is there dummy variable in the data.frame; c = 0 when there is none; c = 1 when there is
#' @param l : number of labeling starts at (default = 1)
#' @examples #ploth(sp500,0,20) for histograms of sp500 which does not has dummy variables
ploth <- function(x,c,l = 1){
typeofvar <- sapply(x,class)
ha <- typeofvar[typeofvar == "numeric"]
if(c == 1){
dumornot <- typeofvar[typeofvar == "integer"]
dummyvar <- x[,c(names(dumornot))]
dummyvar <- as.data.frame(dummyvar[!unique(dummyvar) == 0 & !unique(dummyvar) == 1])
if(dim(dummyvar)[1] > 0){
ha <- c(dumornot,ha)}
}
x <- x[,c(names(ha))]
var <- names(x)
n <- length(var)
for(i in 1:n){
hist(x[,i], main = paste("Fig.", paste(l+i-1, paste("Histogram of",var[i]))), xlab = var[i])
}
}
<file_sep>/R/annu.pv.df.R
##################################################################################################
### Deffered Annuity Present Value
##################################################################################################
#' @name annu.pv.df
#' @aliases annu.pv.df
#' @title Calculate present value of annuity
#' @description Calculate present value of an ordinary annuity or an annuity due.
#' @usage annu.pv.df(pmt,i,n,k)
#' @param pmt :the equal amount of payment of each period
#' @param i :interest rate accoding to the period
#' @param n :number of periods
#' @param k :number of periods deffered
#' @examples #annu.pv(100,0.0248,10,4,0)
annu.pv.df <- function(pmt,i,n,k){
pv <- pmt*((1-((1+i)^-n))/i)*((1+i)^-k)
round(pv,2)
return(pv)
}
<file_sep>/R/bond.price.R
##################################################################################################
### Plain Vanilla Bond
##################################################################################################
#' @name bond.price
#' @aliases bond.price
#' @title Calculate the plain vanilla bond price
#' @description Calculate the plain vanilla bond price
#' @usage bond.price(par,c,n,yield,m)
#' @param par :the face value of the bond
#' @param c :the annual coupon rate of the bond
#' @param n :number of years
#' @param yield :the annual yield to maturity of a bond
#' @param m :couponding period in a year
#' @examples #bond.price(1000,0.03,10,0.0248,2)
bond.price <- function(par,c,n,yield,m){
pmt <- c*par/m
p <- pmt*((1-((1+yield/m)^(-m*n)))/(yield/2))+(par/((1+yield/m)^(m*n)))
return(p)
}
| 29b89b3b8aa7ce730c37e086242cf7bcfd26e4f1 | [
"R"
] | 17 | R | cran/FinAna | 599fc65d1a398c91c6e6ca68555e522c299a3524 | 54a9fa34f48c0e00637d296f4d2423d300482864 | |
refs/heads/master | <repo_name>iamleohanstudy/han<file_sep>/src/test/java/han/handatatest.java
package han;
import han.repository.JdbcRepository;
import junit.framework.TestCase;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.test.annotation.Rollback;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
import org.springframework.test.context.transaction.TransactionConfiguration;
import org.springframework.transaction.annotation.Transactional;
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration("classpath:testdata-config.xml")
@Transactional
public class handatatest extends TestCase {
@Autowired
private JdbcRepository jdbcRepository;
//test
@Test
public void testData1(){
String sqlchange ="update t_account set name ='leohan' where number = 123456789";
jdbcRepository.updateData(sqlchange);
String sql1 = "select name from t_account where number = 123456789";
String result = jdbcRepository.getData(sql1);
assertEquals("leohan", result);
}
@Test
public void testData2(){
String sql1 = "select name from t_account where number = 123456789";
String result = jdbcRepository.getData(sql1);
assertEquals("Keith and <NAME>", result);
}
}
<file_sep>/src/main/java/han/repository/JdbcRepository.java
package han.repository;
public interface JdbcRepository {
public String getData(String sql);
public void updateData(String sql);
}
<file_sep>/src/main/java/han/repository/JdbcRepositoryImpl.java
package han.repository;
import javax.sql.DataSource;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.stereotype.Repository;
import org.springframework.transaction.annotation.Transactional;
@Repository
public class JdbcRepositoryImpl implements JdbcRepository {
private DataSource dataSource;
private JdbcTemplate jdbcTemplate;
@Autowired
JdbcRepositoryImpl(DataSource dataSource){
this.dataSource = dataSource;
this.jdbcTemplate = new JdbcTemplate(dataSource);
}
@SuppressWarnings("deprecation")
@Override
public String getData(String sql) {
String result = jdbcTemplate.queryForObject(sql, String.class);
System.out.println("query result is :"+result);
return result;
}
@Override
public void updateData(String sql) {
// TODO Auto-generated method stub
jdbcTemplate.update(sql);
}
}
<file_sep>/pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>leo</groupId>
<artifactId>han</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>han</name>
<url>http://maven.apache.org</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.11</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>3.2.4.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>3.2.4.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-test</artifactId>
<version>3.2.4.RELEASE</version>
</dependency>
<dependency>
<groupId>hsqldb</groupId>
<artifactId>hsqldb</artifactId>
<version>1.8.0.10</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<configuration>
<source>1.7</source>
<target>1.7</target>
<showDeprecation>true</showDeprecation>
<showWarnings>true</showWarnings>
<executable>${env.JAVA_HOME_7}/bin/javac</executable>
<fork>true</fork>
</configuration>
</plugin>
</plugins>
</build>
</project>
| ab7813fcd426e5d9c9faf8cbb25f72602f371f86 | [
"Java",
"Maven POM"
] | 4 | Java | iamleohanstudy/han | 3db74df63e4446acfca9084ae34a40233610ce99 | 01237a79e3d63e80c1cc95223fef6ab8424c5013 | |
refs/heads/master | <file_sep>version: '3.3'
services:
redis:
image: redis
ports:
- '6379:6379'
db:
restart: always
image: postgres:latest
container_name: mlflow_db
expose:
- ${DB_PORT}
environment:
- POSTGRES_DB=${DB_NAME}
- POSTGRES_USER=${DB_USER}
- POSTGRES_PASSWORD=${DB_PW}
volumes:
- db_datapg:/var/lib/postgresql/data
mlflow:
restart: always
build: ./mlflow
image: mlflow_server
container_name: mlflow_server
ports:
- 5000:5000
environment:
- BACKEND=postgresql://${DB_USER}:${DB_PW}@db:${DB_PORT}/${DB_NAME}
- ARTIFACTS=/mlruns
volumes:
- mlrun_data:/mlruns
command:
- sh
- -c
- mlflow server
--port ${MLFLOW_PORT}
--host 0.0.0.0
--backend-store-uri $${BACKEND}
--default-artifact-root $${ARTIFACTS}
depends_on:
- db
celery:
build: ./celery
volumes:
- ./celery:/celery
depends_on:
- redis
app:
build: ./app
volumes:
- ./app:/app
ports:
- 9091:8000
depends_on:
- celery
expose:
- 9091
volumes:
db_datapg:
mlrun_data:
<file_sep>from celery import Celery
from celery.result import AsyncResult
from flask import (
Flask,
make_response,
render_template,
request,
)
celery_app = Celery('tasks', backend='redis://redis', broker='redis://redis')
app = Flask(__name__)
@app.route('/iris', methods=['GET', 'POST'])
def iris_handler():
if request.method == 'POST':
sl = request.form['sl']
sw = request.form['sw']
pl = request.form['pl']
pw = request.form['pw']
sample = [[int(sl), int(sw), int(pl), int(pw)]]
task = celery_app.send_task('tasks.predict', sample)
return render_template('check.html', value=task.id)
@app.route('/iris/<task_id>', methods=['GET', 'POST'])
def iris_check_handler(task_id):
task = AsyncResult(task_id, app=celery_app)
if task.ready():
labels = {
0: 'iris-setosa',
1: 'iris-versicolor',
2: 'iris-virginica',
}
text = f'DONE, result {labels[int(task.result[0])]}'
else:
text = 'IN_PROGRESS'
response = make_response(text, 200)
response.mimetype = "text/plain"
return response
@app.route('/')
def home():
return render_template('index.html')
if __name__ == '__main__':
app.run(debug=True, host='0.0.0.0', port=8000)
<file_sep>FROM python:3.7
ADD . /celery
WORKDIR /celery
RUN pip install -r requirements.txt
CMD celery -A tasks worker --loglevel=info
<file_sep>import joblib
import mlflow
from sklearn.datasets import load_iris
from sklearn.pipeline import Pipeline
from xgboost import XGBClassifier
from sklearn.base import (
BaseEstimator,
TransformerMixin,
)
class ModelTransformer(TransformerMixin, BaseEstimator):
def __init__(self, mdl):
self.mdl = mdl
def fit(self, X, y=None):
return self.mdl.fit(X, y)
def transform(self, X):
return self.mdl.predict(X)
class JSONResponse(TransformerMixin, BaseEstimator):
def __init__(self, key_name):
self.key_name = key_name
def fit(self, X, y=None):
return X
def transform(self, X):
result = {self.key_name: X[0]}
return result
def predict(self, X):
return self.transform(X)
if __name__ == '__main__':
MLFLOW_SERVER_URL = 'http://127.0.0.1:5000/'
data = load_iris()
params = {
"learning_rate": 0.05,
"n_estimators": 1000,
"max_depth": 3,
"objective": 'multi:softmax',
"num_class": 3,
}
mdl = XGBClassifier(**params)
mdl.fit(data['data'], data['target'])
mdl_api = Pipeline([("mdl", ModelTransformer(mdl)), ("pack", JSONResponse('iris'))])
joblib.dump(mdl, 'iris.mdl')
with mlflow.start_run(run_name='Experiment 1'):
mlflow.sklearn.log_model(mdl_api, 'prod_mdl')
<file_sep>FROM python:3.7
ENV MLFLOW_HOME /opt/mlflow
ENV SERVER_PORT 5000
ENV SERVER_HOST 0.0.0.0
ENV FILE_STORE ${MLFLOW_HOME}/fileStore
ENV ARTIFACT_STORE ${MLFLOW_HOME}/artifactStore
RUN pip install mlflow psycopg2 && \
mkdir -p ${MLFLOW_HOME}/scripts && \
mkdir -p ${FILE_STORE} && \
mkdir -p ${ARTIFACT_STORE}
EXPOSE ${SERVER_PORT}/tcp
WORKDIR ${MLFLOW_HOME}
<file_sep># LSML2
## LSML2 final project by <NAME> 08.09.2021
Dataset: https://scikit-learn.org/stable/modules/generated/sklearn.datasets.load_iris.html
Project goals: Create an ML-service to solve any kind of task.
### Architecture:
- Model: trained on iris dataset XGBClassifier model
### Containers:
1) app: Flask web server to serve simple web interface
2) celery: Celery worker to asynchronously serve model
3) redis: For storing celery tasks
4) mlflow: MLFlow for tracking models
5) db: Database server for mlflow
### Installing:
1) Docker https://docs.docker.com/engine/install/
2) Docker compose https://docs.docker.com/compose/install/
4) To train model run: `pip install -r requirements.txt`, then `python train.py`
### Running:
`sudo docker-compose up --build`
##### Web interface:
User Interface - `http://localhost:9091/`
MLFLow web interface - `http://127.0.0.1:5000/#/`
<file_sep>amqp==5.0.6
billiard==3.6.4.0
cached-property==1.5.2
celery==5.1.2
click==7.1.2
click-didyoumean==0.0.3
click-plugins==1.1.1
click-repl==0.2.0
Flask==2.0.1
importlib-metadata==4.8.1
itsdangerous==2.0.1
Jinja2==3.0.1
kombu==5.1.0
MarkupSafe==2.0.1
prompt-toolkit==3.0.20
pytz==2021.1
redis
six==1.16.0
typing-extensions==3.10.0.2
vine==5.0.0
wcwidth==0.2.5
Werkzeug==2.0.1
zipp==3.5.0
scikit-learn~=0.24.2
xgboost~=1.4.2
mlflow<file_sep>import joblib
import numpy as np
from celery import Celery
celery_app = Celery('tasks', backend='redis://redis', broker='redis://redis')
mdl = joblib.load('iris.mdl')
@celery_app.task(name='tasks.predict')
def predict(data):
result = mdl.predict(np.array([data])).tolist()
return result
| e033c142e9b694a3ad78b576565b55b28c903bc7 | [
"YAML",
"Markdown",
"Python",
"Text",
"Dockerfile"
] | 8 | YAML | damu4/lsml | 761db560802e05a80a8e6b1b0219df0b9440a447 | cc81652d9e0b456e520dfbf9f5bc4b390d5c6685 | |
refs/heads/main | <file_sep>/*
프로그램 설명 : 메일 슬롯 Sender
*/
#include <stdio.h>
#include <tchar.h>
#include <Windows.h>
#define SLOT_NAME _T("\\\\.\\mailslot\\mailbox")
int _tmain(int argc, TCHAR* argv[])
{
HANDLE hMailSlot;
TCHAR message[50];
DWORD bytesWritten;
hMailSlot = CreateFile(
SLOT_NAME,
GENERIC_WRITE,
FILE_SHARE_READ,
NULL,
OPEN_EXISTING,
FILE_ATTRIBUTE_NORMAL,
NULL
);
if (hMailSlot == INVALID_HANDLE_VALUE)
{
_fputts(_T("Unalbe to create mailslot! \n"), stdout);
return 1;
}
while (1)
{
_fputts(_T("MY CMD >"), stdout);
_fgetts(message, sizeof(message) / sizeof(TCHAR), stdin);
if (!WriteFile(hMailSlot, message, _tcslen(message) * sizeof(TCHAR), &bytesWritten, NULL))
{
_fputts(_T("Unable to write!"), stdout);
CloseHandle(hMailSlot);
return 1;
}
if (!_tcscmp(message, _T("exit")))
{
_fputts(_T("Good Bye!"), stdout);
break;
}
}
CloseHandle(hMailSlot);
return 0;
}<file_sep>/*
단순 출력 프로그램
*/
#include <stdio.h>
#include <tchar.h>
#include <windows.h>
int _tmain2(int argc, TCHAR* argv[])
{
SetPriorityClass(GetCurrentProcess(), HIGH_PRIORITY_CLASS);
// GetCurrentProcess() : 현재 실행되고 있는 프로세스 함수를 호출한 프로세스의 핸들을 얻을때 사용하는 함수
// 반환값을 통해 핸들을 얻게 된다. ( 커널 오브젝트의 핸들(정수))
while (1)
{
for (DWORD i = 0; i < 10000; i++)
for (DWORD i = 0; i < 10000; i++);
_fputts(_T("Operation2.exe \n"), stdout);
}
return 0;
}<file_sep>#include <stdio.h>
#include <tchar.h>
#include <windows.h>
int _tmain0(int argc, TCHAR* argvp[])
{
STARTUPINFO si = { 0, };
PROCESS_INFORMATION pi;
si.cb = sizeof(si);
TCHAR command[] = _T("Operation2.exe");
CreateProcess
(
NULL, command , NULL , NULL,
TRUE , 0, NULL , NULL, &si, &pi
);
while (1)
{
for (DWORD i = 0; i < 10000; i++)
for (DWORD i = 0; i < 10000; i++);
_fputts(_T("Operation1.exe \n"), stdout);
}
return 0;
}<file_sep>/*
프로그램 설명 : 프로그램 실행 결과에 따른 반환값 확인
*/
#include <stdio.h>
#include <tchar.h>
#include <Windows.h>
int _tmain(int argc, TCHAR* argv[])
{
STARTUPINFO si = { 0, };
PROCESS_INFORMATION pi;
DWORD state;
si.cb = sizeof(si);
si.dwFlags = STARTF_USEPOSITION | STARTF_USESIZE;
si.dwX = 100;
si.dwY = 200;
si.dwXSize = 300;
si.dwYSize = 200;
si.lpTitle = _T("return & exit");
TCHAR command[] = _T("OperationStateChild.exe");
CreateProcess(NULL, command, NULL, NULL, TRUE,
CREATE_NEW_CONSOLE,
NULL, NULL,
&si, &pi);
for (DWORD i = 0; i < 10000; i++)
for (DWORD i = 0; i < 10000; i++);
GetExitCodeProcess(pi.hProcess, &state);
if (state == STILL_ACTIVE)
_tprintf(_T("STILL_ACTIVE \n\n"));
else
_tprintf(_T("state: %d \n\n"), state);
return 0;
}<file_sep>/*
프로그램 설명 : 명령 프로프트 2차
*/
#include <stdio.h>
#include <tchar.h>
#include <Windows.h>
int CmdProcessing(void)
{
_fputts(_T("Best command prompt>> "), stdout);
//_getts_s(cmdString);
}
<file_sep>/*
프로그램 설명 : 프로그램 실행결과에 따른 반환값
*/
#include <stdio.h>
#include <stdlib.h>
#include <tchar.h>
#include <Windows.h>
int _tmain(int argc, TCHAR* argv[])
{
float num1, num2;
_fputts(_T("Return Value Test \n"), stdout);
_tscanf(_T("%f %f"), &num1, &num2);
if (num2 == 0)
{
exit(-1); // or return -1;
}
_tprintf(_T("Operation Result : %f \n"), num1 / num2);
return 1;
}<file_sep>/*
프로그램 설명
1~10 까지 덧셈하여 출력
기존에 지니던 문제점 해결
*/
#include <stdio.h>
#include <tchar.h>
#include <Windows.h>
int _tmain(int argc, TCHAR* argv[])
{
STARTUPINFO si1 = { 0, };
STARTUPINFO si2 = { 0, };
PROCESS_INFORMATION pi1;
PROCESS_INFORMATION pi2;
DWORD return_val1;
DWORD return_val2;
TCHAR command1[] = _T("PartAdder.exe 1 5");
TCHAR command2[] = _T("PartAdder.exe 6 10");
DWORD sum = 0;
si1.cb = sizeof(si1);
si2.cb = sizeof(si2);
CreateProcess(NULL, command1,
NULL, NULL, TRUE,
0, NULL, NULL,
&si1, &pi1);
CreateProcess(NULL, command2,
NULL, NULL, TRUE,
0, NULL, NULL,
&si2, &pi2);
CloseHandle(pi1.hThread);
CloseHandle(pi2.hThread);
/**********문제 해결을 위해 추가되는 두줄의 코드 ****************/
WaitForSingleObject(pi1.hProcess, INFINITE);
WaitForSingleObject(pi2.hProcess, INFINITE);
GetExitCodeProcess(pi1.hProcess, &return_val1);
GetExitCodeProcess(pi2.hProcess, &return_val2);
if (return_val1 == -1 || return_val2 == -1)
return -1; //비정상적 종료
sum += return_val1;
sum += return_val2;
_tprintf(_T("total: %d \n"), sum);
CloseHandle(pi1.hProcess);
CloseHandle(pi2.hProcess);
return 0;
}
<file_sep>/*
프로그램 설명 : 커널 오브젝트 공유 예제
*/
#include <stdio.h>
#include <tchar.h>
#include <Windows.h>
int _tmain1(int argc, TCHAR* argv[])
{
STARTUPINFO si = { 0, };
PROCESS_INFORMATION pi;
si.cb = sizeof(si);
TCHAR command[] = _T("Operation2.exe");
CreateProcess
(
NULL, command, NULL, NULL, TRUE , 0,
NULL, NULL, &si , &pi
);
DWORD timing = 0;
while (1)
{
for (DWORD i = 0; i < 10000; i++)
for (DWORD i = 0; i < 10000; i++);
_fputts(_T("Parent \n"), stdout);
timing += 1;
if (timing == 2)
SetPriorityClass(pi.hProcess, NORMAL_PRIORITY_CLASS);
}
return 0;
}<file_sep>/*
프로그램 설명 : closeHandle 함수 이해
*/
#include <stdio.h>
#include <tchar.h>
#include <Windows.h>
int _tmain(int argc, TCHAR* argvp[])
{
STARTUPINFO si = { 0, };
PROCESS_INFORMATION pi;
si.cb = sizeof(si);
TCHAR command[] = _T("KernelObjProb2.exe");
CreateProcess(
NULL, command, NULL, NULL, TRUE,
0, NULL, NULL, &si, &pi
);
CloseHandle(pi.hProcess);
return 0;
} | 48adbf4fe90275db17fca65ac194204c3a8b783b | [
"C++"
] | 9 | C++ | MOMO320/WindowsSystemProgram | fe9f33b5eae4d506785e10a555a305998d015b82 | 28f62ad44b4f32342af83f592deab85363706c65 | |
refs/heads/master | <repo_name>KAllan357/telephone<file_sep>/README.md
# Telephone
A simple REST client application that is intentionally missing features. This application is meant to be
worked on in conjunction with an [Answering Machine](https://github.com/KAllan357/answering-machine)
# Instructions
1. Fill in the Dockerfile
2. Fix the FIXME's in `telephone.rb`
# The Goal
To learn various Dockerfile commands:
* FROM, MAINTAINER
* RUN
* ADD
* CMD
<file_sep>/telephone.rb
require 'httparty'
# FIXME
sender = "Telemarketer Bob"
# FIXME
message = "I have a credit card offer you can't refuse."
# FIXME
url = "http://localhost:8080/messages"
json = { from: sender, body: message }
response = HTTParty.post(url, body: JSON.generate(json))
<file_sep>/Dockerfile
# See the reference doc - http://docs.docker.com/reference/builder/
# FROM - what is the base image for this Dockerfile? http://docs.docker.com/reference/builder/#from
# MAINTAINER - who owns this Dockerfile? http://docs.docker.com/reference/builder/#maintainer
# Install Ruby - perhaps from a package manager? http://docs.docker.com/reference/builder/#run
# Install the 'httparty' gem - how do you install gems? http://docs.docker.com/reference/builder/#run
# ADD the telephone(.rb) application - http://docs.docker.com/reference/builder/#add
# Write a CMD - http://docs.docker.com/reference/builder/#cmd
| cabde88b103b4381dc3387778d04f1dbaba76396 | [
"Markdown",
"Ruby",
"Dockerfile"
] | 3 | Markdown | KAllan357/telephone | d6f8183eb27e5b9addd618d0335da3059e162f77 | ffeb4cb3e759d5209d0c7821aa005a5e13e160f7 | |
refs/heads/master | <file_sep>use glium::texture::{DepthFormat, DepthTexture2d, MipmapsOption};
use openxr as xr;
use std::ffi::{c_void, CString};
use std::mem;
use std::os::raw::c_int;
use std::ptr;
use std::ptr::null_mut;
use x11::{glx, glx::arb, xlib};
const GL_SRGB8_ALPHA8: u32 = 0x8C43;
type GlXcreateContextAttribsArb = unsafe extern "C" fn(
dpy: *mut xlib::Display,
fbc: glx::GLXFBConfig,
share_context: glx::GLXContext,
direct: xlib::Bool,
attribs: *const c_int,
) -> glx::GLXContext;
pub struct Backend {
context: glx::GLXContext,
display: *mut xlib::Display,
visual: *mut xlib::XVisualInfo,
fb_config: *mut glx::GLXFBConfig,
drawable: x11::xlib::Drawable,
dimmensions: (u32, u32),
}
impl Backend {
pub fn new() -> Self {
let mut fbcount = 0;
let attr = [
glx::GLX_RGBA,
glx::GLX_DEPTH_SIZE,
24,
glx::GLX_DOUBLEBUFFER,
0,
];
let visual_attribs = [0];
let context_attribs = [
arb::GLX_CONTEXT_MAJOR_VERSION_ARB,
3,
arb::GLX_CONTEXT_MINOR_VERSION_ARB,
0,
0,
];
unsafe {
let c_proc_name = CString::new("glXCreateContextAttribsARB").unwrap();
let proc_addr = glx::glXGetProcAddress(c_proc_name.as_ptr() as *const u8);
let glx_create_context_attribs =
mem::transmute::<_, GlXcreateContextAttribsArb>(proc_addr);
let display = xlib::XOpenDisplay(ptr::null());
let root = xlib::XDefaultRootWindow(display);
let visual = glx::glXChooseVisual(display, 0, attr.as_ptr() as *mut _);
let fb_config = glx::glXChooseFBConfig(
display,
xlib::XDefaultScreen(display),
visual_attribs.as_ptr(),
&mut fbcount,
);
let context = glx_create_context_attribs(
display,
*fb_config,
null_mut(),
xlib::True,
&context_attribs[0] as *const c_int,
);
if context.is_null() {
panic!("glXCreateContextAttribsARB failed")
}
glx::glXMakeCurrent(display, root, context);
Self {
context,
display,
visual,
fb_config,
drawable: root,
dimmensions: (800, 600),
}
}
}
pub unsafe fn xr_session_create_info(&self) -> xr::opengl::SessionCreateInfo {
let visualid = { *self.visual }.visualid as u32;
xr::opengl::SessionCreateInfo::Xlib {
x_display: self.display as *mut _,
glx_fb_config: *self.fb_config as *mut _,
glx_drawable: self.drawable,
visualid: visualid,
glx_context: self.context as *mut _,
}
}
}
impl Drop for Backend {
fn drop(&mut self) {
unsafe {
x11::xlib::XFree(self.fb_config as *mut _);
x11::xlib::XFree(self.visual as *mut _);
x11::xlib::XCloseDisplay(self.display);
}
}
}
unsafe impl glium::backend::Backend for Backend {
fn swap_buffers(&self) -> Result<(), glium::SwapBuffersError> {
unsafe {
x11::glx::glXSwapBuffers(self.display, self.drawable);
}
Ok(())
}
unsafe fn get_proc_address(&self, symbol: &str) -> *const c_void {
let addr = CString::new(symbol.as_bytes()).unwrap();
let addr = addr.as_ptr();
let proc_addr = glx::glXGetProcAddressARB(addr as *const _);
match proc_addr {
Some(proc_addr) => proc_addr as *const _,
_ => ptr::null(),
}
}
fn get_framebuffer_dimensions(&self) -> (u32, u32) {
self.dimmensions
}
fn is_current(&self) -> bool {
true
}
unsafe fn make_current(&self) {
glx::glXMakeCurrent(self.display, self.drawable, self.context);
}
}
pub struct OpenXR {
pub entry: xr::Entry,
pub instance: xr::Instance,
pub session: xr::Session<xr::OpenGL>,
pub resolution: (u32, u32),
predicted_display_time: xr::Time,
frame_stream: xr::FrameStream<xr::OpenGL>,
swap_chain: Option<xr::Swapchain<xr::OpenGL>>,
}
impl OpenXR {
fn new(backend: &mut Backend) -> Self {
let entry = xr::Entry::linked();
let extensions = entry
.enumerate_extensions()
.expect("Cannot enumerate extensions");
let app_info = xr::ApplicationInfo::new().application_name("OpenXR example");
if !extensions.khr_opengl_enable {
panic!("XR: OpenGL extension unsupported");
}
let extension_set = xr::ExtensionSet {
khr_opengl_enable: true,
..Default::default()
};
let instance = entry.create_instance(app_info, &extension_set).unwrap();
let instance_props = instance.properties().expect("Cannot load instance props");
println!(
"loaded instance: {} v{}",
instance_props.runtime_name, instance_props.runtime_version
);
let system = instance
.system(xr::FormFactor::HEAD_MOUNTED_DISPLAY)
.unwrap();
let info = unsafe { backend.xr_session_create_info() };
let (session, frame_stream) = unsafe { instance.create_session(system, &info).unwrap() };
session
.begin(xr::ViewConfigurationType::PRIMARY_STEREO)
.unwrap();
let view_configuration_views = instance
.enumerate_view_configuration_views(system, xr::ViewConfigurationType::PRIMARY_STEREO)
.unwrap();
let resolution = (
view_configuration_views[0].recommended_image_rect_width,
view_configuration_views[0].recommended_image_rect_height,
);
backend.dimmensions = resolution;
Self {
entry,
instance,
session,
frame_stream,
resolution,
predicted_display_time: xr::Time::from_raw(0),
swap_chain: None,
}
}
pub fn update(&mut self) {
let mut buffer = xr::EventDataBuffer::new();
while let Some(event) = self.instance.poll_event(&mut buffer).unwrap() {
use xr::Event::*;
match event {
SessionStateChanged(session_change) => match session_change.state() {
xr::SessionState::RUNNING => {
if self.swap_chain.is_none() {
self.create_swapchain()
}
}
_ => {}
},
_ => {
println!("unhandled event");
}
}
}
}
pub fn create_swapchain(&mut self) {
let swapchain_formats = self.session.enumerate_swapchain_formats().unwrap();
if !swapchain_formats.contains(&GL_SRGB8_ALPHA8) {
panic!("XR: Cannot use OpenGL GL_SRGB8_ALPHA8 swapchain format");
}
let swapchain_create_info: xr::SwapchainCreateInfo<xr::OpenGL> = xr::SwapchainCreateInfo {
create_flags: xr::SwapchainCreateFlags::EMPTY,
usage_flags: xr::SwapchainUsageFlags::COLOR_ATTACHMENT
| xr::SwapchainUsageFlags::SAMPLED,
format: GL_SRGB8_ALPHA8,
sample_count: 1,
width: self.resolution.0,
height: self.resolution.1,
face_count: 1,
// Use array size 2 if you want stereo rendering(See: https://github.com/TheHellBox/SlashMania/wiki/Render-different-image-for-each-eye)
array_size: 2,
mip_count: 1,
};
self.swap_chain = Some(
self.session
.create_swapchain(&swapchain_create_info)
.unwrap(),
);
}
pub fn frame_stream_begin(&mut self) {
let state = self.frame_stream.wait().unwrap();
self.predicted_display_time = state.predicted_display_time;
self.frame_stream.begin().unwrap();
}
pub fn get_swapchain_image(&mut self) -> Option<u32> {
let swapchain = self.swap_chain.as_mut()?;
let images = swapchain.enumerate_images().unwrap();
let image_id = swapchain.acquire_image().unwrap();
swapchain.wait_image(xr::Duration::INFINITE).unwrap();
let image = images[image_id as usize];
Some(image)
}
pub fn frame_stream_end(&mut self) {
let swap_chain = self.swap_chain.as_ref().unwrap();
let eye_rect = xr::Rect2Di {
offset: xr::Offset2Di { x: 0, y: 0 },
extent: xr::Extent2Di {
width: self.resolution.0 as i32,
height: self.resolution.1 as i32,
},
};
let time = self.predicted_display_time;
let left_subimage: xr::SwapchainSubImage<xr::OpenGL> = openxr::SwapchainSubImage::new()
.swapchain(swap_chain)
.image_array_index(0)
.image_rect(eye_rect);
let right_subimage: xr::SwapchainSubImage<xr::OpenGL> = openxr::SwapchainSubImage::new()
.swapchain(swap_chain)
.image_array_index(1)
.image_rect(eye_rect);
let projection_view_left =
xr::CompositionLayerProjectionView::new().sub_image(left_subimage);
let projection_view_right =
xr::CompositionLayerProjectionView::new().sub_image(right_subimage);
let proj_views = [projection_view_left, projection_view_right];
let projection = xr::CompositionLayerProjection::new().views(&proj_views);
self.frame_stream
.end(time, xr::EnvironmentBlendMode::OPAQUE, &[&projection])
.unwrap();
}
pub fn release_swapchain_image(&mut self) {
let swapchain = self.swap_chain.as_mut().unwrap();
swapchain.release_image().unwrap();
}
}
fn main() {
use glium::Surface;
let mut backend = Backend::new();
let mut open_xr = OpenXR::new(&mut backend);
let context =
unsafe { glium::backend::Context::new(backend, false, Default::default()) }.unwrap();
let depthtexture = DepthTexture2d::empty_with_format(
&context,
DepthFormat::F32,
MipmapsOption::EmptyMipmaps,
open_xr.resolution.0,
open_xr.resolution.1,
)
.unwrap();
loop {
open_xr.update();
let swapchain_image = open_xr.get_swapchain_image();
if let Some(swapchain_image) = swapchain_image {
open_xr.frame_stream_begin();
let texture_array = unsafe {
glium::texture::srgb_texture2d_array::SrgbTexture2dArray::from_id(
&context,
glium::texture::SrgbFormat::U8U8U8U8,
swapchain_image,
false,
glium::texture::MipmapsOption::NoMipmap,
glium::texture::Dimensions::Texture2dArray {
width: open_xr.resolution.0,
height: open_xr.resolution.1,
array_size: 2,
},
)
};
let texture_left = texture_array.layer(0).unwrap().mipmap(0).unwrap();
let texture_right = texture_array.layer(1).unwrap().mipmap(0).unwrap();
let mut target_left = glium::framebuffer::SimpleFrameBuffer::with_depth_buffer(
&context,
texture_left,
&depthtexture,
)
.unwrap();
let mut target_right = glium::framebuffer::SimpleFrameBuffer::with_depth_buffer(
&context,
texture_right,
&depthtexture,
)
.unwrap();
target_left.clear_color_and_depth((0.6, 0.0, 0.0, 1.0), 1.0);
target_right.clear_color_and_depth((0.0, 0.0, 0.6, 1.0), 1.0);
open_xr.release_swapchain_image();
open_xr.frame_stream_end();
}
}
}
<file_sep>use std::{marker::PhantomData, mem, ops::Deref};
use crate::*;
#[repr(transparent)]
pub struct CompositionLayer<'a, G: Graphics> {
_inner: sys::CompositionLayerBaseHeader,
_marker: PhantomData<&'a G>,
}
macro_rules! setters {
($ident:ident { $($field:ident : $ty:ty,)* }) => {
impl<'a, G: Graphics> $ident<'a, G> {
$(
pub fn $field(mut self, value: $ty) -> Self {
self.inner.$field = value;
self
}
)*
}
}
}
macro_rules! layer {
($ident:ident { $($field:ident : $ty:ty,)* }) => {
#[derive(Copy, Clone)]
#[repr(transparent)]
pub struct $ident<'a, G: Graphics> {
inner: sys::$ident,
_marker: PhantomData<&'a G>,
}
impl<'a, G: Graphics> $ident<'a, G> {
pub fn new() -> Self {
Self {
inner: sys::$ident {
ty: sys::$ident::TYPE,
..unsafe { mem::zeroed() }
},
_marker: PhantomData,
}
}
pub fn space(mut self, value: &'a Space) -> Self {
self.inner.space = value.as_raw();
self
}
}
setters!($ident {
layer_flags: CompositionLayerFlags,
$($field : $ty,)*
});
impl<'a, G: Graphics> Deref for $ident<'a, G> {
type Target = CompositionLayer<'a, G>;
fn deref(&self) -> &Self::Target {
unsafe { mem::transmute(&self.inner) }
}
}
}
}
layer!(CompositionLayerProjection {});
impl<'a, G: Graphics> CompositionLayerProjection<'a, G> {
pub fn views(mut self, views: &'a [CompositionLayerProjectionView<'a, G>]) -> Self {
assert!(views.len() <= u32::max_value() as usize);
self.inner.view_count = views.len() as u32;
self.inner.views = views.as_ptr() as _;
self
}
}
#[derive(Copy, Clone)]
#[repr(transparent)]
pub struct CompositionLayerProjectionView<'a, G: Graphics> {
inner: sys::CompositionLayerProjectionView,
_marker: PhantomData<&'a G>,
}
impl<'a, G: Graphics> CompositionLayerProjectionView<'a, G> {
pub fn new() -> Self {
Self {
inner: sys::CompositionLayerProjectionView {
ty: sys::CompositionLayerProjectionView::TYPE,
..unsafe { mem::zeroed() }
},
_marker: PhantomData,
}
}
pub fn sub_image(mut self, value: SwapchainSubImage<'a, G>) -> Self {
self.inner.sub_image = sys::SwapchainSubImage {
swapchain: value.swapchain.as_raw(),
image_rect: value.image_rect,
image_array_index: value.image_array_index,
};
self
}
pub fn depth_info_khr(mut self, value: &'a CompositionLayerDepthInfoKHR<'a, G>) -> Self {
self.inner.next = &value.inner as *const _ as _;
self
}
}
setters!(CompositionLayerProjectionView {
pose: Posef,
fov: Fovf,
});
#[derive(Copy, Clone)]
#[repr(transparent)]
pub struct CompositionLayerDepthInfoKHR<'a, G: Graphics> {
inner: sys::CompositionLayerDepthInfoKHR,
_marker: PhantomData<&'a G>,
}
impl<'a, G: Graphics> CompositionLayerDepthInfoKHR<'a, G> {
pub fn new() -> Self {
Self {
inner: sys::CompositionLayerDepthInfoKHR {
ty: sys::CompositionLayerDepthInfoKHR::TYPE,
..unsafe { mem::zeroed() }
},
_marker: PhantomData,
}
}
pub fn sub_image(mut self, value: SwapchainSubImage<'a, G>) -> Self {
self.inner.sub_image = sys::SwapchainSubImage {
swapchain: value.swapchain.as_raw(),
image_rect: value.image_rect,
image_array_index: value.image_array_index,
};
self
}
}
setters!(CompositionLayerDepthInfoKHR {
min_depth: f32,
max_depth: f32,
near_z: f32,
far_z: f32,
});
layer!(CompositionLayerQuad {
eye_visibility: EyeVisibility,
pose: Posef,
size: Vector2f,
});
impl<'a, G: Graphics> CompositionLayerQuad<'a, G> {
pub fn sub_image(mut self, value: SwapchainSubImage<'a, G>) -> Self {
self.inner.sub_image = sys::SwapchainSubImage {
swapchain: value.swapchain.as_raw(),
image_rect: value.image_rect,
image_array_index: value.image_array_index,
};
self
}
}
layer!(CompositionLayerCubeKHR {
eye_visibility: EyeVisibility,
image_array_index: u32,
orientation: Quaternionf,
offset: Vector3f,
});
impl<'a, G: Graphics> CompositionLayerCubeKHR<'a, G> {
pub fn swapchain(mut self, value: &'a Swapchain<G>) -> Self {
self.inner.swapchain = value.as_raw();
self
}
}
layer!(CompositionLayerCylinderKHR {
eye_visibility: EyeVisibility,
pose: Posef,
radius: f32,
central_angle: f32,
aspect_ratio: f32,
});
impl<'a, G: Graphics> CompositionLayerCylinderKHR<'a, G> {
pub fn sub_image(mut self, value: SwapchainSubImage<'a, G>) -> Self {
self.inner.sub_image = sys::SwapchainSubImage {
swapchain: value.swapchain.as_raw(),
image_rect: value.image_rect,
image_array_index: value.image_array_index,
};
self
}
}
layer!(CompositionLayerEquirectKHR {
eye_visibility: EyeVisibility,
pose: Posef,
offset: Vector3f,
scale: Vector2f,
bias: Vector2f,
});
impl<'a, G: Graphics> CompositionLayerEquirectKHR<'a, G> {
pub fn sub_image(mut self, value: SwapchainSubImage<'a, G>) -> Self {
self.inner.sub_image = sys::SwapchainSubImage {
swapchain: value.swapchain.as_raw(),
image_rect: value.image_rect,
image_array_index: value.image_array_index,
};
self
}
}
#[cfg(test)]
mod tests {
use super::*;
#[allow(dead_code)]
fn deref_tricks<G: Graphics>(sess: &Session<G>) {
unsafe {
sess.end_frame(
Time::from_raw(0),
EnvironmentBlendMode::OPAQUE,
&[
&CompositionLayerProjection::new().views(&[]),
&CompositionLayerQuad::new(),
],
)
.unwrap();
}
}
}
| 8d176e03c8cc1871444b62ba1d8655976b2451ca | [
"Rust"
] | 2 | Rust | TheHellBox/openxrs | 600843d97b8e8601713c97ca3cd5b24839277fc5 | 9e328ccf0d04815a261ba244a6cfbf816fba6363 | |
refs/heads/master | <repo_name>B3noit/RENDU_DEFI_F2-M1<file_sep>/proto/niveau2.c
#include "niveau2.h"
<file_sep>/proto/niveau2.h
#ifndef NIVEAU2_H_INCLUDED
#define NIVEAU2_H_INCLUDED
#define WINDOW_WIDTH 1440
#define WINDOW_LENGTH 810
#include "transition1.h"
#endif // NIVEAU2_H_INCLUDED
<file_sep>/proto/introduction.c
#include "introduction.h"
int introduction(tGame *Space)
{
//Creation de quelques couleurs SDL, normale qu'il y est des warnings si on ne les utilises pas.
SDL_Color black = {0,0,0,255}; //noire
SDL_Color white = {255,255,255,255}; //blanc
SDL_Color grey = {60,60,60,255}; //blanc
SDL_Color red = {255,0,0,255}; //rouge
SDL_Color green = {0,255,0,255}; //vert
SDL_Color blue = {0,0,255,255}; //bleu
//Tableau de caractère des chemins de nos images, CF le répertoire des images.
char cSprite[100][100]={"./Assets/Images/darkSpace.png","./Assets/Images/comptoirePrimo.png"};
tSprite Primo;//On déclare un sprite avec lequel le joueur peut interagir,
setFullSprite(&Primo, 900, 394, 416,416, cSprite[1], 0);//on l'initialise.
while(Space->nGameState==1)//Grosse boucle du jeu qui tourne tant que le joueur ne quitte pas.
{
affichSpriteDecor(Space,cSprite,0,0,0,WINDOW_WIDTH,WINDOW_LENGTH);
affichSprite(Space,&Primo);
//On vérifie que le joueur joue et qu'il ne souhaite pas quitter la fenêtre.
handleEvents(Space);
testClicked(&Primo);
if (Primo.nClicked == 1)
{
return 1;
}
//On affiche tout
SDL_RenderPresent(Space->pRenderer);
SDL_RenderClear(Space->pRenderer);//Ecoute, moi du futur, j'ai la solution pour le lag dégeulasse quand le tete est affiché, je cré une variable pour le SDL delay de mon render,
//quand je veux afficher du texte il faut le mettent le nombre de tic voulu et bim pb règlé
}
return 0;
}
| ec23afb0ec9e82c79c2236e40e63aa5b5fb709fb | [
"C"
] | 3 | C | B3noit/RENDU_DEFI_F2-M1 | a7a74bb035ba9337f41730e8b1fddbaaab887bfd | 8be6adb747aab5c45b9ab55a62a59359b880896e | |
refs/heads/master | <file_sep><?php
namespace App\Http\Controllers\Gestionnaire;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
use App\User;
use App\Models\Alert;
use App\Models\Produit;
use App\Models\Statistique;
use App\Charts\TestChart;
use App\Helpers\Helper;
use App\Models\Client;
use App\Models\Fournisseur;
use App\Models\Atelier;
use DB;
class StatistiquesController extends Controller{
public function indexRetour($year){
$lineChart = new TestChart;
$camembertChart = new TestChart;
$i = 0;
$totalRetour = array();
$color = array();
$color[0] = '#00ED96';
$color[1]= '#F1BE25';
$color[2]= '#444BF8';
$backgroundColor = array();
$backgroundColor[0] ="rgba(0, 237, 150,0.7)";
$backgroundColor[1] ="rgba(241, 190, 37,0.8)";
$backgroundColor[2] ="rgba(68, 75, 248,0.8)";
$lineChart->labels(Helper::getMonthsAbv());
$lineChart->options([
'legend'=> [
'labels' => [
'usePointStyle' => true,
'fontFamily' => 'Poppins',
'fontColor' => 'black'
],
],
'tooltips' => [
'enabled' => true,
'mode'=> 'index',
'titleFontSize'=> 12,
'titleFontColor'=> '#000',
'bodyFontColor'=> '#000',
'backgroundColor'=> '#fff',
'titleFontFamily'=> 'Poppins',
'bodyFontFamily'=> 'Poppins',
'cornerRadius'=> 3,
'intersect'=> false,
],
'scales' => [
'xAxes' => [[
'display' => true,
'gridLines' => [
'display' => false,
'drawBorder' => true
],
'scaleLabel' => [
'fontColor' => 'black',
'display' => true,
'labelString' => 'Mois',
'fontSize' => 18,
]
]],
'yAxes' => [[
'display' => true,
'gridLines' => [
'display' => false,
'drawBorder' => true
],
'scaleLabel' => [
'fontColor' => 'black',
'display' => true,
'fontSize' => 18,
'labelString' => "Quantité d'articles"
]
]],
]
]);
foreach (Helper::getTypesRetour() as $type) {
$lineChart->dataset($type , 'line', Statistique::retourParMois($type,$year))->options([
'fill' => 'true',
'borderColor' => '#00ed96',
'backgroundColor' => 'transparent',
'borderColor' => $color[$i],
'borderWidth' => 3,
'pointStyle' => 'circle',
'pointRadius' => 5,
'pointBorderColor' => 'transparent',
'pointBackgroundColor' => $color[$i],
]);
$totalRetour[$i] = Statistique::retourTotalParType($type,$year);
$i++;
}
$camembertChart->labels(Helper::getTypesRetour());
$camembertChart->dataset('Retours' , 'pie', $totalRetour)->options([
'fill' => 'true',
'fontColor' => 'black',
'backgroundColor' => $color,
'hoverBackgroundColor' => $backgroundColor,
]);
$camembertChart->options([
'scales' => [
'xAxes' => [[
'display' => false,
]],
'yAxes' => [[
'display' => false,
]],
]
]);
$yearDisplayed = $year;
return view('quality.statistiques.indexR',compact('lineChart','camembertChart','yearDisplayed'));
}
public function indexArticle($year){
$barChart = new TestChart;
$camembertChart = new TestChart;
$i = 0;
$total = array();
$color = array();
$color[0] = '#00ED96';
$color[1]= '#F1BE25';
$color[2]= '#444BF8';
$backgroundColor = array();
$backgroundColor[0] ="rgba(0, 237, 150,0.7)";
$backgroundColor[1] ="rgba(241, 190, 37,0.8)";
$backgroundColor[2] ="rgba(68, 75, 248,0.8)";
$articles = Produit::all();
$barChart->labels($articles->pluck('nom'));
$barChart->options([
'legend'=> [
'display' => false,
],
'tooltips' => [
'enabled' => true,
'mode'=> 'index',
'titleFontSize'=> 12,
'titleFontColor'=> '#000',
'bodyFontColor'=> '#000',
'backgroundColor'=> '#fff',
'titleFontFamily'=> 'Poppins',
'bodyFontFamily'=> 'Poppins',
'cornerRadius'=> 3,
'intersect'=> false,
],
'scales' => [
'xAxes' => [[
'display' => true,
'gridLines' => [
'display' => false,
'drawBorder' => true
],
'scaleLabel' => [
'fontColor' => 'black',
'display' => true,
'fontSize' => 18,
'labelString' => 'Articles'
]
]],
'yAxes' => [[
'display' => true,
'gridLines' => [
'display' => false,
'drawBorder' => true
],
'scaleLabel' => [
'fontColor' => 'black',
'display' => true,
'fontSize' => 18,
'labelString' => "Nombre"
]
]],
]
]);
$barChart->dataset('Retour par Article' , 'bar', Statistique::retourParArticle($articles,$year))->options([
'fill' => 'true',
'backgroundColor' => '#00ED96',
'borderColor' => '#00ED96',
'borderWidth' => 3,
]);
$typeArticles = Helper::getTypesArticle();
$camembertChart->labels($typeArticles);
$camembertChart->dataset("Retour par Type d'Article" , 'doughnut', Statistique::retourParTypeArticle($typeArticles,$year))->options([
'fill' => 'true',
'fontColor' => 'black',
'backgroundColor' => $color,
'hoverBackgroundColor' => $backgroundColor,
]);
$camembertChart->options([
'scales' => [
'xAxes' => [[
'display' => false,
]],
'yAxes' => [[
'display' => false,
]],
]
]);
$yearDisplayed = $year;
return view('quality.statistiques.indexArticle',compact('camembertChart','barChart','yearDisplayed'));
}
public function indexRetourClient($year){
// retour client par produit ou article
$produits = Produit::where('type', '=' ,'Fini')->get();
$nomsProduit = Produit::where('type', '=' ,'Fini')->pluck('nom');
$nombreretourClient = array();
$i = 0;
foreach($produits as $produit)
{
$nombreretourClientParProduit[$i] = Statistique::retourParArticleType($produit->nom,'Retour client',$year);
$i++ ;
}
$chart = new TestChart;
$chart->labels( $nomsProduit->values() );
//$chart->title('Quantité de retour client par article');
$chart->options([
'legend'=> [
'display' => false,
],
'tooltips' => [
'enabled' => true,
'mode'=> 'index',
'titleFontSize'=> 12,
'titleFontColor'=> '#000',
'bodyFontColor'=> '#000',
'backgroundColor'=> '#fff',
'titleFontFamily'=> 'Poppins',
'bodyFontFamily'=> 'Poppins',
'cornerRadius'=> 3,
'intersect'=> false,
],
'scales' => [
'xAxes' => [[
'display' => true,
'gridLines' => [
'display' => false,
'drawBorder' => true
],
'scaleLabel' => [
'fontColor' => 'black',
'display' => true,
'labelString' => 'Articles',
'fontSize' => 18,
]
]],
'yAxes' => [[
'display' => true,
'gridLines' => [
'display' => false,
'drawBorder' => true
],
'scaleLabel' => [
'fontColor' => 'black',
'display' => true,
'labelString' => "Quantité",
'fontSize' => 18,
]
]],
]
]);
$chart->dataset('Quantité de retour client', 'bar', $nombreretourClientParProduit)->options([
'fill' => 'true',
'borderColor' => '#444BF8',
'backgroundColor' => '#444BF8',
]);;
// retour client par client
$clients = Client::all();
$array = array();
foreach($clients as $client)
{
//$nombreretourClient[$i] = Statistique::retourParClient($client->id);
$array = array_add($array, $client->nom , Statistique::retourParClient($client->id,$year) );
}
array_multisort($array,SORT_DESC); // sort the array
$array2 = array_slice($array, 0, 10, true); // get the first 10 elements only
list($keys, $values) = array_divide($array2); // separate the keys and the values in two arrays
//$nomsClients = Client::all()->pluck('nom');
$chartClient = new TestChart;
$chartClient->labels( $keys );
$chartClient->options([
'legend'=> [
'display' => false,
],
'tooltips' => [
'enabled' => true,
'mode'=> 'index',
'titleFontSize'=> 12,
'titleFontColor'=> '#000',
'bodyFontColor'=> '#000',
'backgroundColor'=> '#fff',
'titleFontFamily'=> 'Poppins',
'bodyFontFamily'=> 'Poppins',
'cornerRadius'=> 3,
'intersect'=> false,
],
'scales' => [
'xAxes' => [[
'display' => true,
'gridLines' => [
'display' => false,
'drawBorder' => true
],
'scaleLabel' => [
'fontColor' => 'black',
'display' => true,
'labelString' => 'Clients',
'fontSize' => 18,
]
]],
'yAxes' => [[
'display' => true,
'gridLines' => [
'display' => false,
'drawBorder' => true
],
'scaleLabel' => [
'fontColor' => 'black',
'display' => true,
'labelString' => "Quantité",
'fontSize' => 18,
]
]],
]
]);
$chartClient->dataset('Quantité de retour client', 'bar', $values)->options([
'fill' => 'true',
'borderColor' => '#444BF8',
'backgroundColor' => '#444BF8',
]);;
$yearDisplayed = $year;
return view('quality.statistiques.indexRC',compact('chart','chartClient','yearDisplayed'));
}
public function indexRetourProduction($year){
// Histogramme 1 : retour production par produit ou article
$produits = Produit::where('type', '=' ,'Fini')->get();
$nomsProduit = Produit::where('type', '=' ,'Fini')->pluck('nom');
$i = 0;
foreach($produits as $produit)
{
$nombreretourProductionParProduit[$i] = Statistique::retourParArticleType($produit->nom,'Retour production',$year);
$i++ ;
}
$chart = new TestChart;
$chart->labels( $nomsProduit->values() );
$chart->options([
'legend'=> [
'display' => false,
],
'tooltips' => [
'enabled' => true,
'mode'=> 'index',
'titleFontSize'=> 12,
'titleFontColor'=> '#000',
'bodyFontColor'=> '#000',
'backgroundColor'=> '#fff',
'titleFontFamily'=> 'Poppins',
'bodyFontFamily'=> 'Poppins',
'cornerRadius'=> 3,
'intersect'=> false,
],
'scales' => [
'xAxes' => [[
'display' => true,
'gridLines' => [
'display' => false,
'drawBorder' => true
],
'scaleLabel' => [
'fontColor' => 'black',
'display' => true,
'labelString' => 'Article',
'fontSize' => 18,
]
]],
'yAxes' => [[
'display' => true,
'gridLines' => [
'display' => false,
'drawBorder' => true
],
'scaleLabel' => [
'fontColor' => 'black',
'display' => true,
'labelString' => "Quantité",
'fontSize' => 18,
]
]],
]
]);
$chart->dataset('Quantité de retour production', 'bar', $nombreretourProductionParProduit)->options([
'fill' => 'true',
'borderColor' => '#F1BE25',
'backgroundColor' => '#F1BE25',
]);;
//Histogramme 2 : retour production par atelier
$ateliers = Atelier::all();
$nomAteliers = Atelier::all()->pluck('nom');
$i = 0;
foreach($ateliers as $atelier)
{
$nombreretourProductionParAtelier[$i] = Statistique::retourParAtelier($atelier->id,$year);
$i++;
}
$chartP = new TestChart;
$chartP->labels( $nomAteliers->values() );
$chartP->options([
'legend'=> [
'display' => false,
],
'tooltips' => [
'enabled' => true,
'mode'=> 'index',
'titleFontSize'=> 12,
'titleFontColor'=> '#000',
'bodyFontColor'=> '#000',
'backgroundColor'=> '#fff',
'titleFontFamily'=> 'Poppins',
'bodyFontFamily'=> 'Poppins',
'cornerRadius'=> 3,
'intersect'=> false,
],
'scales' => [
'xAxes' => [[
'display' => true,
'gridLines' => [
'display' => false,
'drawBorder' => true
],
'scaleLabel' => [
'fontColor' => 'black',
'display' => true,
'labelString' => 'Ateliers',
'fontSize' => 18,
]
]],
'yAxes' => [[
'display' => true,
'gridLines' => [
'display' => false,
'drawBorder' => true
],
'scaleLabel' => [
'fontColor' => 'black',
'display' => true,
'labelString' => "Quantité",
'fontSize' => 18,
]
]],
]
]);
$chartP->dataset('Quantité de retour production', 'bar', $nombreretourProductionParAtelier)->options([
'fill' => 'true',
'borderColor' => '#F1BE25',
'backgroundColor' => '#F1BE25',
]);;
$yearDisplayed = $year;
return view('quality.statistiques.indexRP',compact('chart','chartP','yearDisplayed'));
}
public function indexRetourFournisseur($year){
// Histogramme 1 : retour fournisseur par matiere premiere
$MPs = Produit::where('type', '=' ,'Matiere premiere')->get();
$array = array();
foreach($MPs as $MP)
{
$array = array_add($array, $MP->nom , Statistique::retourParArticleType($MP->nom,'Retour fournisseur',$year) );
}
array_multisort($array,SORT_DESC); // sort the array
$array2 = array_slice($array, 0, 10, true); // get the first 10 elements only
list($keys, $values) = array_divide($array2); // separate the keys and the values in two arrays
$chart = new TestChart;
$chart->labels( $keys );
$chart->options([
'legend'=> [
'display' => false,
],
'tooltips' => [
'enabled' => true,
'mode'=> 'index',
'titleFontSize'=> 12,
'titleFontColor'=> '#000',
'bodyFontColor'=> '#000',
'backgroundColor'=> '#fff',
'titleFontFamily'=> 'Poppins',
'bodyFontFamily'=> 'Poppins',
'cornerRadius'=> 3,
'intersect'=> false,
],
'scales' => [
'xAxes' => [[
'display' => true,
'gridLines' => [
'display' => false,
'drawBorder' => true
],
'scaleLabel' => [
'fontColor' => 'black',
'display' => true,
'labelString' => 'Marchandise',
'fontSize' => 18,
]
]],
'yAxes' => [[
'display' => true,
'gridLines' => [
'display' => false,
'drawBorder' => true
],
'scaleLabel' => [
'fontColor' => 'black',
'display' => true,
'labelString' => "Quantité",
'fontSize' => 18,
]
]],
]
]);
$chart->dataset('Quantité de retour fournisseur', 'bar', $values)->options([
'fill' => 'true',
'borderColor' => '#00ed96',
'backgroundColor' => '#00ed96',
]);;
//Histogramme 2 : retour fournisseur par fournisseur
$fournisseurs = Fournisseur::all();
$array = array();
foreach($fournisseurs as $fournisseur)
{
$array = array_add($array, $fournisseur->nom , Statistique::retourParFournisseur($fournisseur->id,$year) );
}
array_multisort($array,SORT_DESC); // sort the array
$array2 = array_slice($array, 0, 10, true); // get the first 10 elements only
list($keys, $values) = array_divide($array2); // separate the keys and the values in two arrays
$chartF = new TestChart;
$chartF->labels( $keys );
$chartF->options([
'legend'=> [
'display' => false,
],
'tooltips' => [
'enabled' => true,
'mode'=> 'index',
'titleFontSize'=> 12,
'titleFontColor'=> '#000',
'bodyFontColor'=> '#000',
'backgroundColor'=> '#fff',
'titleFontFamily'=> 'Poppins',
'bodyFontFamily'=> 'Poppins',
'cornerRadius'=> 3,
'intersect'=> false,
],
'scales' => [
'xAxes' => [[
'display' => true,
'gridLines' => [
'display' => false,
'drawBorder' => true
],
'scaleLabel' => [
'fontColor' => 'black',
'display' => true,
'labelString' => 'Fournisseurs',
'fontSize' => 18,
]
]],
'yAxes' => [[
'display' => true,
'gridLines' => [
'display' => false,
'drawBorder' => true
],
'scaleLabel' => [
'fontColor' => 'black',
'display' => true,
'labelString' => "Quantité",
'fontSize' => 18,
]
]],
]
]);
$chartF->dataset('Quantité de retour fournisseur', 'bar', $values)->options([
'fill' => 'true',
'borderColor' => '#00ed96',
'backgroundColor' => '#00ed96',
]);;
$yearDisplayed = $year;
return view('quality.statistiques.indexRF',compact('chart','chartF','yearDisplayed'));
}
public function indexAudit($year){
$barChart = new TestChart;
$camembertChart = new TestChart;
$i = 0;
$total = array();
$color = array();
$color[0] = '#F12559';
$color[1]= '#00ED96';
$barChart->labels(Helper::getMonthsAbv());
$barChart->options([
'legend'=> [
'labels' => [
'usePointStyle' => true,
'fontFamily' => 'Poppins',
'fontColor' => 'black'
],
],
'tooltips' => [
'enabled' => true,
'mode'=> 'index',
'titleFontSize'=> 12,
'titleFontColor'=> '#000',
'bodyFontColor'=> '#000',
'backgroundColor'=> '#fff',
'titleFontFamily'=> 'Poppins',
'bodyFontFamily'=> 'Poppins',
'cornerRadius'=> 3,
'intersect'=> false,
],
'scales' => [
'xAxes' => [[
'display' => true,
'gridLines' => [
'display' => false,
'drawBorder' => true
],
'scaleLabel' => [
'fontColor' => 'black',
'display' => true,
'fontSize' => 18,
'labelString' => 'Mois'
]
]],
'yAxes' => [[
'display' => true,
'gridLines' => [
'display' => false,
'drawBorder' => true
],
'scaleLabel' => [
'fontColor' => 'black',
'display' => true,
'fontSize' => 18,
'labelString' => "Nombre"
]
]],
]
]);
foreach (Helper::getResultatAudit() as $resultat) {
$barChart->dataset($resultat , 'bar', Statistique::auditParMois($resultat,$year))->options([
'fill' => 'true',
'backgroundColor' => $color[$i],
'borderColor' => $color[$i],
'borderWidth' => 3,
]);
$total[$i] = Statistique::auditTotalParResulat($resultat,$year);
$i++;
}
$camembertChart->labels(Helper::getResultatAudit());
$camembertChart->dataset('Audits' , 'pie', $total)->options([
'fill' => 'true',
'fontColor' => 'black',
'backgroundColor' => $color,
]);
$camembertChart->options([
'scales' => [
'xAxes' => [[
'display' => false,
]],
'yAxes' => [[
'display' => false,
]],
]
]);
$yearDisplayed = $year;
return view('quality.statistiques.indexA',compact('camembertChart','barChart','yearDisplayed'));
}
public function indexInspection($year){
$histoChart = new TestChart;
$pieChart = new TestChart;
$i = 0;
$total = array();
$color = array();
$color[0] = '#F12559';
$color[1]= '#00ED96';
$histoChart->labels(Helper::getMonthsAbv());
$histoChart->options([
'legend'=> [
'labels' => [
'usePointStyle' => true,
'fontFamily' => 'Poppins',
'fontColor' => 'black'
],
],
'tooltips' => [
'enabled' => true,
'mode'=> 'index',
'titleFontSize'=> 12,
'titleFontColor'=> '#000',
'bodyFontColor'=> '#000',
'backgroundColor'=> '#fff',
'titleFontFamily'=> 'Poppins',
'bodyFontFamily'=> 'Poppins',
'cornerRadius'=> 3,
'intersect'=> false,
],
'scales' => [
'xAxes' => [[
'display' => true,
'gridLines' => [
'display' => false,
'drawBorder' => true
],
'scaleLabel' => [
'fontColor' => 'black',
'display' => true,
'fontSize' => 18,
'labelString' => 'Mois'
]
]],
'yAxes' => [[
'display' => true,
'gridLines' => [
'display' => false,
'drawBorder' => true
],
'scaleLabel' => [
'fontColor' => 'black',
'display' => true,
'fontSize' => 18,
'labelString' => "Nombre"
]
]],
]
]);
foreach (Helper::getTypeInspection() as $resultat) {
$histoChart->dataset($resultat , 'bar', Statistique::inspectionParMois($resultat,$year))->options([
'fill' => 'true',
'backgroundColor' => $color[$i],
'borderColor' => $color[$i],
'borderWidth' => 3,
]);
$total[$i] = Statistique::inspectionTotalParResulat($i,$year);
$i++;
}
$pieChart->labels(Helper::getTypeInspection());
$pieChart->dataset('Inspection' , 'pie', $total)->options([
'fill' => 'true',
'fontColor' => 'black',
'backgroundColor' => $color,
]);
$pieChart->options([
'scales' => [
'xAxes' => [[
'display' => false,
]],
'yAxes' => [[
'display' => false,
]],
]
]);
$yearDisplayed = $year;
return view('quality.statistiques.indexI',compact('histoChart','pieChart','yearDisplayed'));
}
}
<file_sep><?php
use Illuminate\Database\Seeder;
use Carbon\Carbon;
class PermissionsTableSeeder extends Seeder
{
/**
* Run the database seeds.
*
* @return void
*/
public function run()
{
DB::table('permissions')->insert([
'name' => 'edit user',
'guard_name' => 'web',
'description' => 'droit de modification des utilisateurs, privileges et roles',
]);
DB::table('permissions')->insert([
'name' => 'view user',
'guard_name' => 'web',
'description' => 'droit de voir la liste des utilisateurs',
]);
DB::table('permissions')->insert([
'name' => 'edit atelier',
'guard_name' => 'web',
'description' => 'droit de modification des ateliers',
]);
DB::table('permissions')->insert([
'name' => 'edit fournisseur',
'guard_name' => 'web',
'description' => 'droit de modification des fournisseurs',
]);
DB::table('permissions')->insert([
'name' => 'edit client',
'guard_name' => 'web',
'description' => 'droit de modification des clients',
]);
}
}
<file_sep><?php
namespace App\Helpers;
class Helper{
public static function getJours(){
return array(
1 => 'Dimanche',
2 => 'Lundi',
3 => 'Mardi',
4 => 'Mercredi',
5 => 'Jeudi',
6 => 'Vendredi',
7 => 'Samedi',
);
}
public static function getTypesRetour(){
return array(
0 => 'Retour fournisseur',
1 => 'Retour production',
2 => 'Retour client',
);
}
public static function getTypesArticle(){
return array(
0 => 'Fini',
1 => 'Semi-fini',
2 => 'Matiere premiere',
);
}
public static function getMonths(){
return array(
1 => 'Janvier',
2 => 'Février',
3 => 'Mars',
4 => 'Avril',
5 => 'Mai',
6 => 'Juin',
7 => 'Juillet',
8 => 'Août',
9 => 'Septembre',
10 => 'Octobre',
11 => 'Novembre',
12 => 'Décembre',
);
}
public static function getMonthsAbv(){
return array(
0 => 'Jan',
1 => 'Fév',
2 => 'Mar',
3 => 'Avr',
4 => 'Mai',
5 => 'Jui',
6 => 'Jul',
7 => 'Aoû',
8 => 'Sep',
9 => 'Oct',
10 => 'Nov',
11 => 'Déc',
);
}
public static function getResultatAudit(){
return array(
0 => 'Procédé non conforme',
1 => 'Procédé conforme',
);
}
public static function getResultatInspection(){
return array(
0 => 'Les réponses des examens ne sont pas toutes correctes',
1 => 'Les réponses des examens sont toutes correctes',
);
}
public static function getTypeInspection(){
return array(
0 => 'Echoué',
1 => 'Réussie',
);
}
}
?><file_sep><?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Client extends Model
{
protected $fillable = [
'nom','description','adresse',
];
public function anomalies()
{
return $this->hasMany('App\Models\Anomalie');
}
public function alerts()
{
return $this->hasMany('App\Models\Alert');
}
}
<file_sep><?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
use App\Models\Client;
use App\Models\Produit;
use App\Models\Fournisseur;
use App\Models\Article;
use App\Models\Audit;
use App\Models\Anomalie;
use App\Models\Inspection;
use Carbon\Carbon;
use App\Helpers\Helper;
use Illuminate\Support\Facades\Auth;
class Statistique extends Model
{
/** Accueil **/
static function alerteNonTraite(){
return count(Alert::where("etat","=","nouveau")
->whereNotIn('type',['Rappel'])->where("user_id","=",Auth::user()->id)->get());
}
static function anomalieEnCours(){
return count(Anomalie::where("etat","=","en cours")->get());
}
static function inspectionEnAttente(){
return count(Inspection::where("etat","=","nouveau")->get());
}
static function auditPlanifie(){
return count(Audit::where("etat","=","nouveau")->get());
}
static function retoursRécents(){
return Alert::where("etat","=","nouveau")->whereNotIn('type',['Rappel'])->orderBy('updated_at','desc')->limit(3)->get();
}
static function auditsRécents(){
return Audit::where("etat","=","nouveau")->orderBy('updated_at','desc')->limit(2)->get();
}
static function inspectionsRécentes(){
return Inspection::where("etat","=","nouveau")->orderBy('updated_at','desc')->limit(2)->get();
}
/** Retours **/
static function findbyType($type,$year){
return Alert::where("type","=",$type)->whereYear('created_at','=',$year);
}
static function retourParMoisType($mois,$year,$type){
$sum = 0;
foreach(Statistique::findbyType($type,$year)->whereMonth('created_at','=',$mois)->get() as $alert){
$sum += $alert->lot->quantite;
}
return $sum;
}
static function retourTotalParType($type,$year){
$sum = 0;
foreach(Statistique::findbyType($type,$year)->get() as $alert){
$sum += $alert->lot->quantite;
}
return $sum;
}
static function retourParMois($type,$year){
$retour = array();
foreach(Helper::getMonthsAbv() as $mois){
$month = array_search($mois,Helper::getMonthsAbv());
$monthNum = sprintf("%02s",$month+1);
$retour[$month] = Statistique::retourParMoisType($monthNum,$year,$type);
}
return $retour;
}
/** Retour par critere **/
// retour par critere client
static function retourParClient($id,$year){
$sum = 0;
$alerts = Alert::where("type","=",'Retour client')
->whereYear('created_at','=',$year)->get();// retour client
foreach( $alerts as $alert){
if ($alert->client_id == $id)
{
$sum += $alert->lot->quantite;
}
}
return $sum;
}
// retour par critere atelier
static function retourParAtelier($id,$year){
$sum = 0;
$alerts = Alert::where("type","=",'Retour production')
->whereYear('created_at','=',$year)->get();// retour production
foreach( $alerts as $alert){
if ($alert->atelier_id == $id)
{
$sum += $alert->lot->quantite;
}
}
return $sum;
}
// retour par critere fournisseur
static function retourParFournisseur($id,$year){
$sum = 0;
$alerts = Alert::where("type","=",'Retour fournisseur')
->whereYear('created_at','=',$year)->get();// retour fournisseur
foreach( $alerts as $alert){
if ($alert->fournisseur_id == $id)
{
$sum += $alert->lot->quantite;
}
}
return $sum;
}
/** Retours par article et type **/
static function retourParArticleType($nom,$type,$year){
$sum = 0;
$alerts = Alert::where("type","=",$type)->whereYear('created_at','=',$year)->get();
foreach( $alerts as $alert){
if ($alert->lot->produit->nom == $nom)
{
$sum += $alert->lot->quantite;
}
}
return $sum;
}
/** Retour par Article **/
static function findRetourByArticle($article,$year){
$alerts = Alert::whereYear("created_at","=",$year)->whereNotIn('type',['Rappel'])->get();
$sum = 0;
foreach ($alerts as $alert) {
if($alert->lot->produit->id == $article->id){
$sum += $alert->lot->quantite;
}
}
return $sum;
}
static function retourParArticle($articles, $year){
$retourParArticle = array();
$i = 0;
foreach ($articles as $article) {
$retourParArticle[$i] = Statistique::findRetourByArticle($article,$year);
$i++;
}
return $retourParArticle;
}
static function findRetourByTypeArticle($type,$year){
$alerts = Alert::whereYear("created_at","=",$year)->whereNotIn('type',['Rappel'])->get();
$sum = 0;
foreach ($alerts as $alert) {
if($alert->lot->produit->type == $type){
$sum += $alert->lot->quantite;
}
}
return $sum;
}
static function retourParTypeArticle($types,$year){
$retourParTypeArticle = array();
$i = 0;
foreach ($types as $type) {
$retourParTypeArticle[$i] = Statistique::findRetourByTypeArticle($type,$year);
$i++;
}
return $retourParTypeArticle;
}
static function retourParMoisArticle($mois,$year,$article){
$alerts = Alert::whereYear("created_at","=",$year)->whereNotIn('type',['Rappel'])
->whereMonth("created_at","=",$mois)->get();
$sum = 0;
foreach ($alerts as $alert){
if($alert->lot->produit->id == $article->id){
$sum += $alert->lot->quantite;
}
}
return $sum;
}
/** Audits **/
static function findbyResultatA($resultat,$year){
return Audit::where("resultats","=",$resultat)->where("etat","=","traité")->whereYear('updated_at','=',$year);
}
static function auditParMoisResultat($mois,$year,$resultat){
return count(Statistique::findbyResultatA($resultat,$year)->whereMonth('updated_at','=',$mois)->get());
}
static function auditParMois($resultat,$year){
$audit = array();
foreach(Helper::getMonthsAbv() as $mois){
$month = array_search($mois,Helper::getMonthsAbv());
$monthNum = sprintf("%02s",$month+1);
$audit[$month] = Statistique::auditParMoisResultat($monthNum,$year,$resultat);
}
return $audit;
}
static function auditTotalParResulat($resultat,$year){
return count(Statistique::findbyResultatA($resultat,$year)->get());
}
/** Inspections **/
static function findbyResultatI($resultat,$year){
return Inspection::where("resultats","=",$resultat)->where("etat","=","traité")->whereYear('updated_at','=',$year);
}
static function InspectionParMoisResultat($mois,$year,$typeNum){
$resultat = Helper::getResultatInspection()[$typeNum];
return count(Statistique::findbyResultatI($resultat,$year)->whereMonth('updated_at','=',$mois)->get());
}
static function inspectionTotalParResulat($index,$year){
$resultat = Helper::getResultatInspection()[$index];
return count(Statistique::findbyResultatI($resultat,$year)->get());
}
static function inspectionParMois($resultat,$year){
$inspection = array();
foreach(Helper::getMonthsAbv() as $mois){
$month = array_search($mois,Helper::getMonthsAbv());
$monthNum = sprintf("%02s",$month+1);
$inspection[$month] = Statistique::InspectionParMoisResultat($monthNum,$year,
array_search($resultat,Helper::getTypeInspection()));
}
return $inspection;
}
}
<file_sep><?php
namespace App\Exports;
use App\Models\Client;
use Maatwebsite\Excel\Concerns\Exportable;
use Maatwebsite\Excel\Concerns\FromQuery;
use Maatwebsite\Excel\Concerns\WithHeadings;
use Maatwebsite\Excel\Concerns\ShouldAutoSize;
use Maatwebsite\Excel\Concerns\WithMapping;
use Maatwebsite\Excel\Concerns\WithTitle;
use Maatwebsite\Excel\Concerns\WithEvents;
use Maatwebsite\Excel\Events\AfterSheet;
class ClientsExport implements FromQuery,WithMapping,WithHeadings,ShouldAutoSize,WithTitle,WithEvents
{
use Exportable;
public function map($client): array
{
return [
$client->id,
$client->nom,
$client->adresse,
$client->description,
$client->created_at,
];
}
public function headings(): array
{
return [
'#',
'Nom',
'Adresse',
'Description',
'Créé le',
];
}
public function title(): string
{
return 'Clients';
}
public function query()
{
return Client::query();
}
public function registerEvents(): array{
$styleArray = [
'borders' => [
'allBorders' => [
'borderStyle' => \PhpOffice\PhpSpreadsheet\Style\Border::BORDER_THIN,
],
],
];
return [
AfterSheet::class => function(AfterSheet $event) use($styleArray) {
$cpt = count(Client::all()) +1;
$cellRange = 'A1:E1';
$event->sheet->getStyle($cellRange)->ApplyFromArray($styleArray);
$event->sheet->getDelegate()->getStyle($cellRange)->getFont()->setName('Calibri');
$event->sheet->getDelegate()->getStyle($cellRange)->getFont()->setSize(14);
$event->sheet->getStyle('A2:A'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('B2:B'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('C2:C'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('D2:D'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('E2:E'.$cpt)->applyFromArray($styleArray);
},
];
}
}
<file_sep><?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Alert extends Model
{
public function lot()
{
return $this->belongsTo('App\Models\Lot');
}
public function anomalie()
{
return $this->belongsTo('App\Models\Anomalie');
}
public function event()
{
return $this->belongsTo('App\Models\Event');
}
public function atelier()
{
return $this->belongsTo('App\Models\Atelier');
}
public function client()
{
return $this->belongsTo('App\Models\Client');
}
public function fournisseur()
{
return $this->belongsTo('App\Models\Fournisseur');
}
}
<file_sep><?php
namespace App\Http\Controllers\Gestionnaire;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
use App\Models\Event;
use App\Models\Inspection;
use App\Models\Alert;
use App\Models\Audit;
use Carbon\Carbon;
use Redirect,Response;
use DB;
use Auth;
class CalendrierController extends Controller
{
public function index()
{
if(request()->ajax())
{
$start = (!empty($_GET["start"])) ? ($_GET["start"]) : ('');
$end = (!empty($_GET["end"])) ? ($_GET["end"]) : ('');
$data = Event::whereDate('start', '>=', $start)->whereDate('end', '<=', $end)->get(['id','title','type','start', 'end']);
return Response::json($data);
}
return view('quality.calendrier.calender');
}
public function create(Request $request)
{
$type = $request->type;
$inspection_id = 0;
$audit_id = 0;
$event = new Event;
$event->title = $request->title;
$event->type = $request->type;
$event->start = $request->start;
$event->end = $request->end;
$event->rappel = $request->rappel;
$event->user_id = Auth::user()->id;
if ($type == "Inspection"){
$inspection_id = DB::table('inspections')->insertGetId(
['titre' => $request->title,
'etat' => "nouveau",
'step' => 1,
'created_at' => Carbon::now(),
'updated_at' => Carbon::now(),
]
);
$event->inspection_id = $inspection_id;
}
else{
$audit_id = DB::table('audits')->insertGetId(
['titre' => $request->title,
'etat' => "nouveau",
'step' => 1,
'created_at' => Carbon::now(),
'updated_at' => Carbon::now(), ]
);
$event->audit_id = $audit_id;
}
$event->save();
return Response::json($event);
}
public function update(Request $request)
{
$where = array('id' => $request->id);
$updateArr = ['start' => $request->start, 'end' => $request->end];
$event = Event::where($where)->update($updateArr);
return Response::json($event);
}
public function destroy(Request $request){
$event = Event::where("id","=",$request->id)->delete();
return Response::json($event);
}
}
<file_sep><?php
Auth::routes();
Route::get('/', 'HomeController@index')->middleware('auth','general')->name('home');
/* AlertRF CRUD Routes for simple user */
Route::group([
'as' => 'alertRF.',
'prefix' => 'alertRF',
'namespace'=>'Simple',
'middleware' => ['auth','general', 'can:edit fournisseur']],
function(){
Route::get('create', 'AlertController@createRF')->name('create');
Route::post('create', 'AlertController@storeRF')->name('store');
Route::get('edit/{alert}', 'AlertController@editRF')->name('edit');
Route::get('view/{alert}', 'AlertController@viewRF')->name('view');
Route::post('update/{alert}', 'AlertController@updateRF')->name('update');
Route::delete('delete/{alert}', 'AlertController@deleteRF')->name('delete');
Route::get('fiche/{alert}', 'AlertController@generate_pdf_RF')->name('pdf');
});
/* AlertRP CRUD Routes for simple user */
Route::group([
'as' => 'alertRP.',
'prefix' => 'alertRP',
'namespace'=>'Simple',
'middleware' => ['auth','general', 'can:edit atelier']],
function(){
Route::get('create', 'AlertController@createRP')->name('create');
Route::post('create', 'AlertController@storeRP')->name('store');
Route::get('edit/{alert}', 'AlertController@editRP')->name('edit');
Route::get('view/{alert}', 'AlertController@viewRP')->name('view');
Route::post('update/{alert}', 'AlertController@updateRP')->name('update');
Route::delete('delete/{alert}', 'AlertController@deleteRP')->name('delete');
Route::get('fiche/{alert}', 'AlertController@generate_pdf_RP')->name('pdf');
});
/* AlertRC CRUD Routes for simple user */
Route::group([
'as' => 'alertRC.',
'prefix' => 'alertRC',
'namespace'=>'Simple',
'middleware' => ['auth','general', 'can:edit client']],
function(){
Route::get('create', 'AlertController@createRC')->name('create');
Route::post('create', 'AlertController@storeRC')->name('store');
Route::get('edit/{alert}', 'AlertController@editRC')->name('edit');
Route::get('view/{alert}', 'AlertController@viewRC')->name('view');
Route::post('update/{alert}', 'AlertController@updateRC')->name('update');
Route::delete('delete/{alert}', 'AlertController@deleteRC')->name('delete');
Route::get('fiche/{alert}', 'AlertController@generate_pdf_RC')->name('pdf');
});
/* To make both gestionnaire & simple users view list alerts */
Route::get('alertRF/list', 'Simple\AlertController@showRF')
->middleware('auth', 'general','role_or_permission:edit fournisseur|gestionnaire')
->name('alertRF.list');
Route::get('alertRC/list', 'Simple\AlertController@showRC')
->middleware('auth','general', 'role_or_permission:edit client|gestionnaire')
->name('alertRC.list');
Route::get('alertRP/list', 'Simple\AlertController@showRP')
->middleware('auth','general', 'role_or_permission:edit atelier|gestionnaire')
->name('alertRP.list');
Route::get('alert/update/{alert}', 'Simple\AlertController@updateRead')
->middleware('auth','general')
->name('alert.updateRead');
/********** ADMIN USER Routes ***********/
/* Admin authentication accueil route */
Route::get('admin/accueil',function () { return view('admin.accueil');})
->middleware('auth','general', 'role:admin')
->name('admin.accueil');
/* User CRUD Routes for admin user only */
Route::group([
'as' => 'user.',
'prefix' => 'user',
'namespace'=>'Admin',
'middleware' => ['auth','general', 'role:admin', 'can:edit user']],
function(){
Route::get('dashbord', 'UserController@index')->name('dashbord');
Route::get('create', 'UserController@create')->name('create');
Route::post('create', 'UserController@store')->name('store');
Route::get('edit/{user}', 'UserController@edit')->name('edit');
Route::post('update/{user}', 'UserController@update')->name('update');
Route::delete('delete/{user}', 'UserController@delete')->name('delete');
});
/* Role CRUD Routes for admin user only */
Route::group([
'as' => 'role.',
'prefix' => 'role',
'namespace'=>'Admin',
'middleware' => ['auth', 'general','role:admin', 'can:edit user']],
function(){
Route::get('dashbord', 'RoleController@index')->name('dashbord');
Route::get('create', 'RoleController@create')->name('create');
Route::post('create', 'RoleController@store')->name('store');
Route::get('edit/{role}', 'RoleController@edit')->name('edit');
Route::post('update/{role}', 'RoleController@update')->name('update');
Route::delete('delete/{role}', 'RoleController@delete')->name('delete');
});
/* Permision CRUD Routes for admin user only */
Route::group([
'as' => 'permission.',
'prefix' => 'permission',
'namespace'=>'Admin',
'middleware' => ['auth', 'general','role:admin' , 'can:edit user']],
function(){
Route::get('dashbord', 'PermissionController@index')->name('dashbord');
Route::get('create', 'PermissionController@create')->name('create');
Route::post('create', 'PermissionController@store')->name('store');
Route::get('edit/{permission}', 'PermissionController@edit')->name('edit');
Route::post('update/{permission}', 'PermissionController@update')->name('update');
Route::delete('delete/{permission}', 'PermissionController@delete')->name('delete');
});
/********** Gestionnaire USER Routes ***********/
/* Gestionnaire authentication accueil route */
Route::get('gestionnaire/accueil',function () { return view('gestionnaire.accueil');})
->middleware('auth','general', 'role:gestionnaire')
->name('gestionnaire.accueil');
/* Action CRUD Routes for gestionnaire user */
Route::group([
'as' => 'action.',
'prefix' => 'action',
'namespace'=>'Gestionnaire',
'middleware' => ['auth','general', 'role:gestionnaire']],
function(){
Route::get('list', 'ActionController@index')->name('list');
Route::get('create', 'ActionController@create')->name('create');
Route::post('create', 'ActionController@store')->name('store');
Route::get('edit/{action}', 'ActionController@edit')->name('edit');
Route::post('update/{action}', 'ActionController@update')->name('update');
Route::delete('delete/{action}', 'ActionController@delete')->name('delete');
});
/* Anomalie CRUD Routes for gestionnaire user */
Route::group([
'as' => 'anomalie.',
'prefix' => 'anomalie',
'namespace'=>'Gestionnaire',
'middleware' => ['auth','general', 'role:gestionnaire']],
function(){
Route::get('dashbord', 'AnomalieController@index')->name('dashbord');
Route::get('create', 'AnomalieController@createFromScratch')->name('createFromScratch');
Route::get('create/{id}', 'AnomalieController@createFrom')->name('createFrom');
Route::post('create-step1', 'AnomalieController@postCreateStep1')->name('create-step1');
Route::post('create-step2', 'AnomalieController@postCreateStep2')->name('create-step2');
Route::post('create-step3', 'AnomalieController@postCreateStep3')->name('create-step3');
Route::post('store', 'AnomalieController@store')->name('create-step4');
Route::delete('delete/{anomalie}', 'AnomalieController@delete')->name('delete');
Route::get('previous/{anomalie}', 'AnomalieController@previous')->name('previous');
Route::get('pdf/{anomalie}', 'AnomalieController@generate_pdf')->name('pdf');
Route::get('view/{anomalie}', 'AnomalieController@view')->name('view');
Route::get('export', 'AnomalieController@export')->name('export');
});
/* Inspection CRUD Routes for gestionnaire user */
Route::group([
'as' => 'inspection.',
'prefix' => 'inspection',
'namespace'=>'Gestionnaire',
'middleware' => ['auth', 'general','role:gestionnaire']],
function(){
Route::get('dashbord', 'InspectionController@index')->name('dashbord');
Route::get('create', 'InspectionController@create')->name('create');
Route::get('create/{id}', 'InspectionController@createFrom')->name('createFrom');
Route::post('create-step1', 'InspectionController@postCreateStep1')->name('create-step1');
Route::post('create-step2', 'InspectionController@postCreateStep2')->name('create-step2');
Route::post('store', 'InspectionController@store')->name('create-step3');
Route::delete('delete/{inspection}', 'InspectionController@delete')->name('delete');
Route::get('previous/{inspection}', 'InspectionController@previous')->name('previous');
Route::get('edit/{inspection}', 'InspectionController@edit')->name('edit');
Route::get('pdf/{inspection}', 'InspectionController@generate_pdf')->name('pdf');
Route::get('view/{inspection}', 'InspectionController@view')->name('view');
Route::get('export', 'InspectionController@export')->name('export');
});
/* Audit CRUD Routes for gestionnaire user */
Route::group([
'as' => 'audit.',
'prefix' => 'audit',
'namespace'=>'Gestionnaire',
'middleware' => ['auth','general', 'role:gestionnaire']],
function(){
Route::get('dashbord', 'AuditController@index')->name('dashbord');
Route::get('create', 'AuditController@create')->name('create');
Route::get('create/{id}', 'AuditController@createFrom')->name('createFrom');
Route::post('create-step1', 'AuditController@postCreateStep1')->name('create-step1');
Route::post('create-step2', 'AuditController@postCreateStep2')->name('create-step2');
Route::post('store', 'AuditController@store')->name('create-step3');
Route::delete('delete/{audit}', 'AuditController@delete')->name('delete');
Route::delete('deleteQ/{questionnaire}', 'AuditController@deleteQ')->name('deleteQ');
Route::get('previous/{audit}', 'AuditController@previous')->name('previous');
Route::get('edit/{audit}', 'AuditController@edit')->name('edit');
Route::get('pdf/{audit}', 'AuditController@generate_pdf')->name('pdf');
Route::get('view/{audit}', 'AuditController@view')->name('view');
Route::get('export', 'AuditController@export')->name('export');
});
/* Calendrier CRUD Routes for gestionnaire user */
Route::group([
'as' => 'calendrier.',
'prefix' => 'calendrier',
'namespace'=>'Gestionnaire',
'middleware' => ['auth','general', 'role:gestionnaire']],
function(){
Route::get('/', 'CalendrierController@index')->name('dashbord');
Route::post('/create','CalendrierController@create');
Route::post('/update','CalendrierController@update');
Route::post('/delete','CalendrierController@destroy');
});
/* Test CRUD Routes for gestionnaire user */
Route::group([
'as' => 'test.',
'prefix' => 'test',
'namespace'=>'Gestionnaire',
'middleware' => ['auth','general', 'role:gestionnaire']],
function(){
Route::get('list', 'TestController@show')->name('list');
Route::get('create', 'TestController@create')->name('create');
Route::post('create', 'TestController@store')->name('store');
Route::get('edit/{test}', 'TestController@edit')->name('edit');
Route::post('update/{test}', 'TestController@update')->name('update');
Route::delete('delete/{test}', 'TestController@delete')->name('delete');
Route::get('createExamen', 'TestController@createExamen')->name('createExamen');
Route::post('createExamen','TestController@storeExamen')->name('storeExamen');
});
/* Régle qualité CRUD Routes for gestionnaire user */
Route::group([
'as' => 'regle.',
'prefix' => 'regle',
'namespace'=>'Gestionnaire',
'middleware' => ['auth','general', 'role:gestionnaire']],
function(){
Route::get('list', 'RegleController@show')->name('list');
Route::get('create', 'RegleController@create')->name('create');
Route::post('create', 'RegleController@store')->name('store');
Route::get('edit/{regle}', 'RegleController@edit')->name('edit');
Route::post('update/{regle}', 'RegleController@update')->name('update');
Route::delete('delete/{regle}', 'RegleController@delete')->name('delete');
});
Route::group([
'namespace'=>'Front',
'middleware' => ['auth'] ],
function(){
Route::get('index123', 'UserController@Getindex');
});
/* Statistiques routes */
Route::group([
'as' => 'statistiques.',
'namespace'=>'Gestionnaire',
'prefix' => 'statistiques',
'middleware' => ['auth', 'general','role:gestionnaire|admin']],
function(){
Route::get('retour/{year}', 'StatistiquesController@indexRetour')->name('retour');
Route::get('article/{year}', 'StatistiquesController@indexArticle')->name('article');
Route::get('audit/{year}', 'StatistiquesController@indexAudit')->name('audit');
Route::get('inspection/{year}', 'StatistiquesController@indexInspection')->name('inspection');
Route::get('retourClient/{year}', 'StatistiquesController@indexRetourClient')->name('retourClient');
Route::get('retourFournisseur/{year}', 'StatistiquesController@indexRetourFournisseur')->name('retourFournisseur');
Route::get('retourProduction/{year}', 'StatistiquesController@indexRetourProduction')->name('retourProduction');
});
/********** SIMPLE USER ***********/
/* Simple user authentication accueil route */
Route::get('simple/accueil',function () { return view('simple.accueil');})
->middleware('auth','general', 'role:simple')
->name('simple.accueil');
/* Produit CRUD Routes for simple user */
Route::group([
'as' => 'produit.',
'prefix' => 'produit',
'namespace'=>'Simple',
'middleware' => ['auth','general', 'role:simple']],
function(){
Route::get('list', 'ProduitController@index')->name('list');
Route::get('create', 'ProduitController@create')->name('create');
Route::post('create', 'ProduitController@store')->name('store');
Route::get('edit/{produit}', 'ProduitController@edit')->name('edit');
Route::post('update/{produit}', 'ProduitController@update')->name('update');
Route::delete('delete/{produit}', 'ProduitController@delete')->name('delete');
Route::get('export', 'ProduitController@export')->name('export');
Route::post('import', 'ProduitController@import')->name('import');
});
/* Client CRUD Routes for simple user */
Route::group([
'as' => 'client.',
'prefix' => 'client',
'namespace'=>'Simple',
'middleware' => ['auth', 'general','permission:edit client']],
function(){
Route::get('list', 'ClientController@index')->name('list');
Route::get('create', 'ClientController@create')->name('create');
Route::post('create', 'ClientController@store')->name('store');
Route::get('edit/{client}', 'ClientController@edit')->name('edit');
Route::post('update/{client}', 'ClientController@update')->name('update');
Route::delete('delete/{client}', 'ClientController@delete')->name('delete');
Route::get('export', 'ClientController@export')->name('export');
Route::post('import', 'ClientController@import')->name('import');
});
/* Fournisseur CRUD Routes for simple user */
Route::group([
'as' => 'fournisseur.',
'prefix' => 'fournisseur',
'namespace'=>'Simple',
'middleware' => ['auth','general', 'permission:edit fournisseur']],
function(){
Route::get('list', 'FournisseurController@index')->name('list');
Route::get('create', 'FournisseurController@create')->name('create');
Route::post('create', 'FournisseurController@store')->name('store');
Route::get('edit/{fournisseur}', 'FournisseurController@edit')->name('edit');
Route::post('update/{fournisseur}', 'FournisseurController@update')->name('update');
Route::delete('delete/{fournisseur}', 'FournisseurController@delete')->name('delete');
Route::get('export', 'FournisseurController@export')->name('export');
Route::post('import', 'FournisseurController@import')->name('import');
});
/* Atelier CRUD Routes for simple user */
Route::group([
'as' => 'atelier.',
'prefix' => 'atelier',
'namespace'=>'Simple',
'middleware' => ['auth','general', 'permission:edit atelier']],
function(){
Route::get('list', 'AtelierController@index')->name('list');
Route::get('create', 'AtelierController@create')->name('create');
Route::post('create', 'AtelierController@store')->name('store');
Route::get('edit/{atelier}', 'AtelierController@edit')->name('edit');
Route::post('update/{atelier}', 'AtelierController@update')->name('update');
Route::delete('delete/{atelier}', 'AtelierController@delete')->name('delete');
Route::get('export', 'AtelierController@export')->name('export');
Route::post('import', 'AtelierController@import')->name('import');
});
<file_sep><?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreateAuditsTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('audits', function (Blueprint $table) {
$table->bigIncrements('id');
$table->text('titre');
$table->text('description')->nullable();
$table->integer('step')->default(1);
$table->enum('etat', ['nouveau','en cours','traité'])->default('nouveau');
$table->text('resultats')->nullable();
$table->text('commentaire')->nullable();
$table->string('productimg')->nullable();
$table->unsignedBigInteger('user_id')->nullable();
$table->unsignedBigInteger('atelier_id')->nullable();
$table->unsignedBigInteger('procede_id')->nullable();
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
/*Schema::table('alerts', function (Blueprint $table) {
$table->dropForeign('alerts_event_id_foreign');
$table->dropColumn('event_id');
$table->dropForeign('alerts_anomalie_id_foreign');
$table->dropColumn('anomalie_id');
}); */
Schema::dropIfExists('audits');
}
}
<file_sep><?php
namespace App\Imports;
use App\Models\Produit;
use Maatwebsite\Excel\Concerns\ToModel;
use Maatwebsite\Excel\Concerns\WithHeadingRow;
class ProduitsImport implements ToModel,WithHeadingRow
{
/**
* @param array $row
*
* @return \Illuminate\Database\Eloquent\Model|null
*/
public function model(array $row)
{
return new Produit([
'nom' => $row['nom'],
'modele' => $row['modele'],
'reference' => $row['reference'],
'description' => $row['description'],
'type' => $row['type'],
'prix' => $row['prix'],
]);
}
}
<file_sep><?php
use Illuminate\Database\Seeder;
class LotsTableSeeder extends Seeder
{
/**
* Run the database seeds.
*
* @return void
*/
public function run()
{
DB::table('lots')->insert([
'id' => '2',
'titre' => '',
'quantite' => '10',
'caracteristiquep' => '2 m',
'produit_id' => '10',
]);
DB::table('lots')->insert([
'id' => '1',
'titre' => '',
'quantite' => '45',
'caracteristiquep' => 'Vert',
'produit_id' => '8',
]);
DB::table('lots')->insert([
'id' => '3',
'titre' => '',
'quantite' => '45',
'caracteristiquep' => 'Rouge',
'produit_id' => '11',
]);
DB::table('lots')->insert([
'id' => '4',
'titre' => '',
'quantite' => '20',
'caracteristiquep' => 'Female',
'produit_id' => '9',
]);
}
}
<file_sep><?php
namespace App\Http\Controllers\Simple;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
use App\Models\Fournisseur;
use App\Exports\FournisseursExport;
use App\Imports\FournisseursImport;
use Maatwebsite\Excel\Facades\Excel;
class FournisseurController extends Controller
{
/**
* @return \Illuminate\Support\Collection
*/
public function export(){
return Excel::download(new FournisseursExport, 'fournisseurs.xlsx');
}
/**
* @return \Illuminate\Support\Collection
*/
public function import(){
Excel::import(new FournisseursImport,request()->file('file'));
return back();
}
public function index(){
$fournisseurs = Fournisseur::paginate(15);
return view('simple.fournisseur.index',compact('fournisseurs'));
}
public function create(){
$actionForm = route('fournisseur.create');
return view('simple.fournisseur.form', compact('actionForm'));
}
public function store(Request $request){
$this->validate($request,[
'nom' => 'required',
'description' => 'required',
'adresse' => 'required'
]);
$fournisseur = new Fournisseur;
$fournisseur->nom = $request->nom;
$fournisseur->description = $request->description;
$fournisseur->adresse = $request->adresse;
$fournisseur->save();
return redirect(route('fournisseur.list'))->with('successMsg',"Le fournisseur est ajouté avec succès");
}
public function edit(Fournisseur $fournisseur){
$actionForm = route('fournisseur.update', ['fournisseur' => $fournisseur]);
return view('simple.fournisseur.form',compact('fournisseur','actionForm'));
}
public function update(Request $request, Fournisseur $fournisseur){
$this->validate($request,[
'nom' => 'required',
'description' => 'required',
'adresse' => 'required'
]);
$fournisseur->nom = $request->nom;
$fournisseur->description = $request->description;
$fournisseur->adresse = $request->adresse;
$fournisseur->save();
return redirect(route('fournisseur.list'))->with('successMsg',"Le fournisseur est modifié avec succès");
}
public function delete(Fournisseur $fournisseur){
$fournisseur->delete();
return redirect(route('fournisseur.list'));
}
}
<file_sep><?php
namespace App\Http\Controllers\Simple;
use DB;
use Illuminate\Database\Eloquent\Model;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
use App\Models\Alert;
use App\Models\Lot;
use App\Models\Produit;
use App\Models\Caracteristique;
use App\Models\Atelier;
use App\Models\Client;
use App\Models\Fournisseur;
use App\Models\Anomalie;
use App\User;
use Illuminate\Database\Seeder;
use Illuminate\Support\Facades\Auth;
use Carbon\Carbon;
use PDF;
class AlertController extends Controller
{
public function updateRead(Request $request, Alert $alert){
$alert->etat = "en cours";
$alert->save();
return back();
}
/*----------------- CRUD alertRF --------------------*/
public function createRF(){
$action = route('alertRF.create');
return view('simple.alert.formRF', compact('action'));
}
public function storeRF(Request $request){
$this->validate($request,[
'fournisseur' => 'required',
'produit' => 'required',
'caracteristiquep' => 'required',
'quantite' => ['required','integer']
]);
$alert = new Alert;
$lot = new Lot;
$alert->type = 'Retour fournisseur';
if(!empty($request->description)) {
$alert->description= $request->description;
}
if(!empty($request->motif)) {
$alert->motif= $request->motif;
}
$alert->fournisseur_id = $request->fournisseur;
$lot_id = DB::table('lots')->insertGetId([
'quantite' => $request->quantite,
'caracteristiquep' => $request->caracteristiquep,
'produit_id' => $request->produit
]);
/* notify the QHSE user */
$users = User::whereHas("roles", function($q){ $q->where("name", "gestionnaire"); })->get();
$alert->user_id = $users[0]->id;
$alert->sent = 0;
$alert->etat = "nouveau";
/* automaticly create an associated anomaly */
$anomalie = new Anomalie;
$anomalie->lot_id = $lot_id;
$anomalie->fournisseur_id = $request->fournisseur;
$anomalie->type = 'Retour fournisseur';
$anomalie->step = 1;
$anomalie->etat = "nouveau";
$anomalie->created_at = Carbon::now();
$anomalie->updated_at = Carbon::now();
if(!empty($request->description)) {
$anomalie->description= $request->description;
}
$anomalie->save();
$alert->anomalie_id = $anomalie->id;
$alert->lot_id = $lot_id;
$alert->save();
if(!empty($request->motif)) {
$anomalie->titre = $alert->motif. ' N°'.$anomalie->id;
}
else{
$anomalie->titre = '';
}
$anomalie->save();
return redirect(route('alertRF.list'))->with('successMsg',"Création d'une nouvelle alerte");
}
public function showRF(){
$alerts = Alert::where('type', '=', 'Retour fournisseur' )->orderBy('id','desc')->paginate(15);
return view('simple.alert.listRF',compact('alerts'));
}
public function editRF(Alert $alert){
$action = route('alertRF.update', ['alert' => $alert]);
return view('simple.alert.formRF',compact('alert','action'));
}
public function updateRF(Request $request, Alert $alert){
$this->validate($request,[
'fournisseur' => 'required',
'produit' => 'required',
'caracteristiquep' => 'required',
'quantite' => ['required','integer'],
]);
if(!empty($request->description)) {
$alert->description= $request->description;
}
else{
$alert->description= '';
}
if(!empty($request->motif)) {
$alert->motif= $request->motif;
}
else{
$alert->motif= '';
}
$alert->fournisseur_id = $request->fournisseur;
$update_lot = DB::table('lots')
->where('id', $alert->lot_id )
->update([
'quantite' => $request->quantite,
'caracteristiquep' => $request->caracteristiquep,
'produit_id' => $request->produit
]);
$anomalie = Anomalie::where('id','=',$alert->anomalie_id)->first();
$anomalie->lot_id = $alert->lot_id;
$anomalie->fournisseur_id = $request->fournisseur_id;
$anomalie->type = 'Retour fournisseur';
$anomalie->step = 1;
$anomalie->etat = "nouveau";
$anomalie->created_at = Carbon::now();
$anomalie->updated_at = Carbon::now();
if(!empty($request->description)) {
$anomalie->description= $request->description;
}
else{
$anomalie->description = '';
}
if(!empty($request->motif)) {
$anomalie->titre = $request->motif. ' N°'.$anomalie->id;
}
else{
$anomalie->titre = '';
}
$anomalie->save();
$alert->save();
return redirect(route('alertRF.list'))->with('successMsg','Modification alerte réussiée');
}
public function deleteRF(Alert $alert){
DB::table('lots')->where('id', $alert->lot_id )->delete();
DB::table('anomalies')->where('id', $alert->anomalie_id )->delete();
$alert->delete();
return redirect(route('alertRF.list'));
}
function generate_pdf_RF(Alert $alert) {
$pdf = PDF::loadView('pdf.ficheRF', compact('alert'));
return $pdf->stream('Fiche.pdf');
}
public function viewRF(Alert $alert){
return view('simple.alert.viewRF',compact('alert'));
}
/*----------------- CRUD alertRC --------------------*/
public function createRC(){
$action = route('alertRC.create');
return view('simple.alert.formRC', compact('action'));
}
public function storeRC(Request $request){
$this->validate($request,[
'client' => 'required',
'caracteristiquep' => 'required',
'quantite' => ['required','integer'],
'produit' => 'required',
]);
$alert = new Alert;
$lot = new Lot;
$alert->type = 'Retour client';
if(!empty($request->description)) {
$alert->description= $request->description;
}
if(!empty($request->motif)) {
$alert->motif= $request->motif;
}
$alert->client_id = $request->client;
/* notify the QHSE user */
$users = User::whereHas("roles", function($q){ $q->where("name", "gestionnaire"); })->get();
$alert->user_id = $users[0]->id;
$alert->sent = 0;
$alert->etat = "nouveau";
$lot_id = DB::table('lots')->insertGetId([
'quantite' => $request->quantite,
'produit_id' => $request->produit ,
'caracteristiquep' => $request->caracteristiquep
]);
/* automaticly create an associated anomaly */
$anomalie = new Anomalie;
$anomalie->lot_id = $lot_id;
$anomalie->client_id = $request->client;
$anomalie->type = 'Retour client';
$anomalie->step = 1;
$anomalie->etat = "nouveau";
$anomalie->created_at = Carbon::now();
$anomalie->updated_at = Carbon::now();
if(!empty($request->description)) {
$anomalie->description= $request->description;
}
$anomalie->save();
$alert->anomalie_id = $anomalie->id;
$alert->lot_id = $lot_id;
$alert->save();
if(!empty($request->motif)) {
$anomalie->titre = $request->motif. ' N°'.$anomalie->id;
}
else{
$anomalie->titre = '';
}
$anomalie->save();
return redirect(route('alertRC.list'))->with('successMsg',"Création d'une nouvelle alerte");
}
public function showRC(){
$alerts = Alert::where('type', '=', 'Retour client' )
->orderBy('id','desc')->paginate(15);
return view('simple.alert.listRC',compact('alerts'));
}
public function editRC(Alert $alert){
$action = route('alertRC.update', ['alert' => $alert]);
return view('simple.alert.formRC',compact('alert','action'));
}
public function updateRC(Request $request, Alert $alert){
$this->validate($request,[
'client' => 'required',
'quantite' => ['required','integer'],
'produit' => 'required',
'caracteristiquep' => 'required'
]);
$alert->type = 'Retour client';
if(!empty($request->description)) {
$alert->description= $request->description;
}
else{
$alert->description= '';
}
if(!empty($request->motif)) {
$alert->motif= $request->motif;
}
else{
$alert->motif= '';
}
$alert->client_id = $request->client;
$alert->sent = 0;
$update_lot = DB::table('lots')
->where('id', $alert->lot_id )
->update(['quantite' => $request->quantite, 'produit_id' => $request->produit ,
'caracteristiquep' => $request->caracteristiquep]);
$anomalie = Anomalie::where('id','=',$alert->anomalie_id)->first();
$anomalie->lot_id = $alert->lot_id;
$anomalie->client_id = $alert->client_id;
$anomalie->type = 'Retour client';
$anomalie->step = 1;
$anomalie->etat = "nouveau";
if(!empty($request->description)) {
$anomalie->description= $request->description;
}
else{
$anomalie->description= '';
}
if(!empty($request->motif)) {
$anomalie->titre = $request->motif. ' N°'.$anomalie->id;
}
else{
$anomalie->titre = '';
}
$anomalie->save();
$alert->save();
return redirect(route('alertRC.list'))->with('successMsg','Modification alerte réussiée');
}
public function deleteRC(Alert $alert){
DB::table('lots')->where('id', $alert->lot_id )->delete();
DB::table('anomalies')->where('id', $alert->anomalie_id )->delete();
$alert->delete();
return redirect(route('alertRC.list'));
}
public function viewRC(Alert $alert){
return view('simple.alert.viewRC',compact('alert'));
}
function generate_pdf_RC(Alert $alert){
$pdf = PDF::loadView('pdf.ficheRC', compact('alert'));
return $pdf->stream('Fiche.pdf');
}
/*----------------- CRUD alertRP --------------------*/
public function createRP(){
$action = route('alertRP.create');
return view('simple.alert.formRP', compact('action'));
}
public function storeRP(Request $request){
$this->validate($request,[
'atelier' => 'required',
'quantite' => ['required','integer'],
'produit' => 'required',
'caracteristiquep' => 'required'
]);
$alert = new Alert;
$lot = new Lot;
$alert->type = 'Retour production';
if(!empty($request->description)) {
$alert->description= $request->description;
}
if(!empty($request->motif)) {
$alert->motif= $request->motif;
}
$alert->atelier_id = $request->atelier;
$lot_id = DB::table('lots')->insertGetId(
['quantite' => $request->quantite, 'produit_id' => $request->produit ,
'caracteristiquep' => $request->caracteristiquep]
);
/* notify the QHSE user */
$users = User::whereHas("roles", function($q){ $q->where("name", "gestionnaire"); })->get();
$alert->user_id = $users[0]->id;
$alert->sent = 0;
$alert->etat = "nouveau";
/* automaticly create an associated anomaly */
$anomalie = new Anomalie;
$anomalie->lot_id = $lot_id;
$anomalie->atelier_id = $request->atelier;
$anomalie->type = 'Retour production';
$anomalie->step = 1;
$anomalie->etat = "nouveau";
$anomalie->created_at = Carbon::now();
$anomalie->updated_at = Carbon::now();
if(!empty($request->description)) {
$anomalie->description= $request->description;
}
$anomalie->save();
$alert->anomalie_id = $anomalie->id;
$alert->lot_id = $lot_id;
$alert->save();
if(!empty($request->motif)) {
$anomalie->titre = $request->motif. ' N°'.$anomalie->id;
}
else{
$anomalie->titre = '';
}
$anomalie->save();
return redirect(route('alertRP.list'))->with('successMsg',"Création d'une nouvelle alerte");
}
public function showRP(){
$alerts = Alert::where('type', '=', 'Retour production' )->orderBy('id','desc')->paginate(15);
return view('simple.alert.listRP',compact('alerts'));
}
public function editRP(Alert $alert){
$action = route('alertRP.update', ['alert' => $alert]);
return view('simple.alert.formRP',compact('alert','action'));
}
public function updateRP(Request $request, Alert $alert){
$this->validate($request,[
'atelier' => 'required',
'quantite' => ['required','integer'],
'produit' => 'required',
'caracteristiquep' => 'required'
]);
$alert->type = 'Retour production';
if(!empty($request->description)) {
$alert->description= $request->description;
}
else{
$alert->description= "";
}
if(!empty($request->motif)) {
$alert->motif= $request->motif;
}
else{
$alert->motif= '';
}
$alert->atelier_id = $request->atelier;
$update_lot = DB::table('lots')
->where('id', $alert->lot_id )
->update(['quantite' => $request->quantite, 'produit_id' => $request->produit ,
'caracteristiquep' => $request->caracteristiquep]);
$anomalie = Anomalie::where('id','=',$alert->anomalie_id)->first();
$anomalie->lot_id = $alert->lot_id;
$anomalie->atelier_id = $request->atelier_id;
$anomalie->type = 'Retour production';
$anomalie->step = 1;
$anomalie->etat = "nouveau";
if(!empty($request->description)) {
$anomalie->description= $request->description;
}
else{
$anomalie->description= '';
}
if(!empty($request->motif)) {
$anomalie->titre = $request->motif. ' N°'.$anomalie->id;
}
else{
$anomalie->titre = '';
}
$anomalie->save();
$alert->save();
return redirect(route('alertRP.list'))->with('successMsg','Modification alerte réussiée');
}
public function deleteRP(Alert $alert){
DB::table('lots')->where('id', $alert->lot_id )->delete();
DB::table('anomalies')->where('id', $alert->anomalie_id )->delete();
$alert->delete();
return redirect(route('alertRP.list'));
}
function generate_pdf_RP(Alert $alert) {
$pdf = PDF::loadView('pdf.ficheRP', compact('alert'));
return $pdf->stream('Fiche.pdf');
}
public function viewRP(Alert $alert){
return view('simple.alert.viewRP',compact('alert'));
}
}
<file_sep><?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreateAlertsTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('alerts', function (Blueprint $table) {
$table->bigIncrements('id');
$table->integer('sent')->default(0);
$table->enum('etat', ['nouveau','en cours', 'traité'])->nullable();
$table->enum('type', ['Rappel','Retour fournisseur', 'Retour client', 'Retour production']);
$table->text('description')->nullable();
$table->text('motif')->nullable();
$table->date('start')->nullable(); //for calendar
$table->unsignedBigInteger('lot_id')->nullable();
$table->unsignedBigInteger('client_id')->nullable();
$table->unsignedBigInteger('fournisseur_id')->nullable();
$table->unsignedBigInteger('atelier_id')->nullable();
$table->unsignedBigInteger('user_id')->nullable(); /* receiver of the alert */
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('alerts');
}
}
<file_sep><?php
namespace App\Http\Middleware;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Auth;
use Spatie\Permission\Models\Role;
use Spatie\Permission\Models\Permission;
use App\User;
use App\Models\Alert;
use App\Models\Event;
use App\Models\Inspection;
use App\Models\Audit;
use App\Helpers\Helper;
use DB;
use Closure;
use Carbon\Carbon;
class GeneralMiddleware
{
/**
* Handle an incoming request.
*
* @param \Illuminate\Http\Request $request
* @param \Closure $next
* @return mixed
*/
public function handle($request, Closure $next){
if(Auth::user()){
$this->deleteEventsRelations();
$this->createEventsAlert();
$this->displayAlerts($request);
}
return $next($request);
}
public function deleteEventsRelations(){
$audits = Audit::all();
foreach ($audits as $audit) {
if( count(Event::where('audit_id','=',$audit->id)->get()) == 0 ){
$audit->delete();
}
}
$inspections = Inspection::all();
foreach ($inspections as $inspection) {
if( count(Event::where('inspection_id','=',$inspection->id)->get()) == 0 ){
$inspection->delete();
}
}
}
public function createEventsAlert(){
$eventsARappeler = Event::where('rappel','=','true')->get(); // reduce the for loop
foreach($eventsARappeler as $event) {
if($event->alert_id == null){
$alert= new Alert;
$alert->type = "Rappel";
$alert->start = $event->start;
$alert->user_id = $event->user_id;
$alert->event_id = $event->id;
$alert->save();
$event->alert_id = $alert->id;
$event->save();
}
elseif($event->alert->start != $event->start){
$event->alert->start = $event->start;
$event->alert->etat= null;
$event->alert->save();
}
}
}
public function displayAlerts(Request $request){
$alerts = Alert::where('user_id','=',Auth::user()->id)
->where('sent','=',0)
->whereNotIn('etat', ["en cours","traité"])
->orWhereNull('etat')->get();
foreach ($alerts as $alert) {
$currentDate = Carbon::now()->toDateString();
$currentDateT = Carbon::parse($currentDate)->timestamp;
$startT = Carbon::parse($alert->start)->timestamp;
$difference = $startT - $currentDateT;
if(($alert->type == "Rappel") && ($difference < 96400) && ($difference > 3600) ) {
$alert->etat= "nouveau";
$alert->save();
}
if($alert->type != "Rappel"){
$alert->sent=1;
$alert->save();
}
}
$toasts = array();
$aF = $alerts->where('type','=','Retour fournisseur');
$aP = $alerts->where('type','=','Retour production');
$aC = $alerts->where('type','=','Retour client');
$toasts = array_add($toasts,0, count($aF));
$toasts = array_add($toasts,1, count($aP));
$toasts = array_add($toasts,2, count($aC));
$request->session()->put('toasts', $toasts);
}
}
<file_sep><?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Produit extends Model
{
protected $fillable = [
'nom' , 'modele', 'reference','description','type','prix'
];
public function caracteristiques()
{
return $this->hasMany('App\Models\Caracteristique');
}
public function procedes()
{
return $this->hasMany('App\Models\Procede');
}
public function lots()
{
return $this->hasMany('App\Models\Lot');
}
public function fichiers()
{
return $this->hasMany('App\Models\Fichier');
}
public function regles()
{
return $this->hasMany('App\Models\Regle');
}
public function audits()
{
return $this->hasMany('App\Models\Audit');
}
}
<file_sep><?php
use Illuminate\Database\Seeder;
class AlertsTableSeeder extends Seeder
{
/**
* Run the database seeds.
*
* @return void
*/
public function run()
{
/* DB::table('alerts')->insert([
'id' => '1',
'sent' => '0',
'etat' => 'nouveau',
'type' => 'Retour fournisseur',
'description' => 'Retour ABS du fournisseur <NAME>',
'motif' => 'Mauvaise qualité de ABS',
'lot_id' => '1',
'fournisseur_id' => '13',
'user_id' => '5',
'anomalie_id' => '1',
'created_at' => '2020-08-15 13:36:36',
'updated_at' => '2020-08-15 13:36:36'
]);
DB::table('alerts')->insert([
'id' => '2',
'sent' => '0',
'etat' => 'nouveau',
'type' => 'Retour fournisseur',
'description' => 'Retour Câble blanc du fournisseur <NAME>',
'motif' => 'Très faible conductivité',
'lot_id' => '2',
'fournisseur_id' => '3',
'user_id' => '5',
'anomalie_id' => '2',
'created_at' => '2020-08-15 13:36:36',
'updated_at' => '2020-08-15 13:36:36'
]);
DB::table('alerts')->insert([
'id' => '3',
'sent' => '0',
'etat' => 'nouveau',
'type' => 'Retour fournisseur',
'description' => 'Retour PVC du fournisseur Bouhoun Pub',
'motif' => 'Faible qualité de PVC',
'lot_id' => '3',
'fournisseur_id' => '4',
'user_id' => '5',
'anomalie_id' => '3',
'created_at' => '2020-08-15 13:36:36',
'updated_at' => '2020-08-15 13:36:36'
]);
DB::table('alerts')->insert([
'id' => '4',
'sent' => '0',
'etat' => 'nouveau',
'type' => 'Retour fournisseur',
'description' => 'Retour 3P plug au fournisseur Aitcom Pub',
'motif' => 'Réception une petite quantité (inférieure à la quantité achetée )',
'lot_id' => '4',
'fournisseur_id' => '2',
'user_id' => '5',
'anomalie_id' => '4',
'created_at' => '2020-08-15 13:36:36',
'updated_at' => '2020-08-15 13:36:36'
]); */
}
}
<file_sep><?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Examen extends Model
{
public function test()
{
return $this->belongsTo('App\Test');
}
public function reponse()
{
return $this->hasOne('App\Models\Reponse');
}
}
<file_sep><?php
use Illuminate\Database\Seeder;
use Carbon\Carbon;
class ActionTableSeeder extends Seeder
{
/**
* Run the database seeds.
*
* @return void
*/
public function run()
{
DB::table('actions')->insert([
'id' => '1',
'type' => 'corrective',
'designation' => 'Intervention service réparation',
'description' => 'Réparation des articles défectueux par les réparateurs',
'resultat' => 'Article mis en état et fonctionnel',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('actions')->insert([
'id' => '2',
'type' => 'corrective',
'designation' => 'Intervention service maintenance',
'description' => 'Maintenance de la machine de production',
'resultat' => 'Machine en bon état de fonctionnement',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('actions')->insert([
'id' => '3',
'type' => 'corrective',
'designation' => 'Arrêter la machine de production',
'description' => 'Arrêt de la machine afin de diagnostiquer et corriger les problèmes et ensuite réparer la machine',
'resultat' => 'Machine de production fonctionnelle',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('actions')->insert([
'id' => '4',
'type' => 'corrective',
'designation' => 'Intervention opérateur machine',
'description' => 'Paramétrage et réglage de la machine de production',
'resultat' => 'Machine de production paramétrée',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('actions')->insert([
'id' => '5',
'type' => 'corrective',
'designation' => 'Instruction et ordres aux ouvriers',
'description' => 'Donner des instructions aux ouvriers pour les orienter vers les bonnes pratiques de travail',
'resultat' => 'Travail rigoureux et satisfaisant',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
}
}
<file_sep><?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Spatie\Permission\Models\Role;
use Spatie\Permission\Models\Permission;
use App\Models\Alert;
use App\Models\Event;
use Illuminate\Support\Facades\Auth;
use DB;
use Carbon\Carbon;
use App\User;
use App\Helpers\Helper;
class HomeController extends Controller
{
/**
* Create a new controller instance.
*
* @return void
*/
public function __construct()
{
$this->middleware('auth');
}
/**
* Show the application dashboard.
*
* @return \Illuminate\Contracts\Support\Renderable
*/
public function index(Request $request)
{
if (Auth::check() && Auth::user()->hasRole('admin')) {
return redirect()->route('user.dashbord');
}
elseif (Auth::check() && Auth::user()->hasRole('simple')) {
return redirect()->route('produit.list');
}
return view('main.accueil');
}
}
<file_sep>function sweetSuccess(successMsg) {
if(successMsg != ''){
swal({
title: "Bravo!",
text: successMsg,
type: "success",
showConfirmButton: true
});
}
}
$('#toastr-warning-bottom-right').click(function() {
toastr.warning('This Is warning Message','Bottom Right',{
"positionClass": "toast-bottom-right",
timeOut: 5000,
"closeButton": true,
"debug": false,
"newestOnTop": true,
"progressBar": true,
"preventDuplicates": true,
"onclick": null,
"showDuration": "300",
"hideDuration": "1000",
"extendedTimeOut": "1000",
"showEasing": "swing",
"hideEasing": "linear",
"showMethod": "fadeIn",
"hideMethod": "fadeOut",
"tapToDismiss": false
})
});
<file_sep><?php
use Illuminate\Database\Seeder;
use Carbon\Carbon;
class AtelierTableSeeder extends Seeder
{
/**
* Run the database seeds.
*
* @return void
*/
public function run()
{
DB::table('ateliers')->insert([
'id' => '1',
'nom' => 'SMT',
'metier' => 'Production des cartes électroniques pour la RAM et flashs disques',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('ateliers')->insert([
'id' => '2',
'nom' => 'Injection',
'metier' => 'Injection plastique pour la souris,chargeurs et flashs disques',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('ateliers')->insert([
'id' => '3',
'nom' => 'Unité Production Câble',
'metier' => 'Production des câbles alimenations et câbles USB',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('ateliers')->insert([
'id' => '4',
'nom' => 'Montage et emballage',
'metier' => 'Monatge des souris et chargeurs ainsi que emballages de tous les produits',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('ateliers')->insert([
'id' => '5',
'nom' => 'Contrôle',
'metier' => 'Test et contrôle des produits en cours de lors fabrication et les produits finis',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
}
}
<file_sep><?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Charge extends Model
{
public function anomalie()
{
return $this->belongsTo('App\Models\Anomalie');
}
}
<file_sep><?php
use Illuminate\Database\Seeder;
use Carbon\Carbon;
class ProduitTableSeeder extends Seeder
{
/**
* Run the database seeds.
*
* @return void
*/
public function run()
{
DB::table('produits')->insert([
'id' => '1',
'nom' => 'Flash disque 2.0',
'type' => 'Fini',
'modele' => 'MANHATTAN',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('produits')->insert([
'id' => '2',
'nom' => 'Flash disque 3.0',
'type' => 'Fini',
'modele' => 'MANHATTAN',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('produits')->insert([
'id' => '3',
'nom' => 'Souris',
'modele' => 'AS47',
'type' => 'Fini',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('produits')->insert([
'id' => '4',
'nom' => 'Chargeur',
'modele' => 'DIAMOND',
'type' => 'Fini',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('produits')->insert([
'id' => '5',
'nom' => 'Câble USB',
'modele' => 'CBL3IP',
'type' => 'Fini',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('produits')->insert([
'id' => '6',
'nom' => '<NAME>',
'modele' => 'CEA',
'type' => 'Fini',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('produits')->insert([
'id' => '7',
'nom' => '<NAME>',
'modele' => 'DDRIII',
'type' => 'Fini',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('produits')->insert([
'id' => '8',
'nom' => 'ABS',
'type' => 'Matiere premiere',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('produits')->insert([
'id' => '9',
'nom' => '3P plug',
'type' => 'Matiere premiere',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('produits')->insert([
'id' => '10',
'nom' => 'Cable blanc',
'type' => 'Matiere premiere',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('produits')->insert([
'id' => '11',
'nom' => 'PVC',
'type' => 'Matiere premiere',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('produits')->insert([
'id' => '12',
'nom' => 'PCBA',
'type' => 'Matiere premiere',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('produits')->insert([
'id' => '13',
'nom' => 'Connecteur USB',
'type' => 'Semi-fini',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('produits')->insert([
'id' => '14',
'nom' => '<NAME>',
'type' => 'Semi-fini',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('produits')->insert([
'id' => '15',
'nom' => 'Connecteur',
'type' => 'Matiere premiere',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('produits')->insert([
'id' => '16',
'nom' => 'Câble',
'type' => 'Matiere premiere',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
}
}
<file_sep><?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Test extends Model
{
public function examens()
{
return $this->hasMany('App\Models\Examen');
}
public function inspections()
{
return $this->hasMany('App\Models\Inspection');
}
}
<file_sep><?php
namespace App\Exports;
use App\Models\Anomalie;
use Maatwebsite\Excel\Concerns\Exportable;
use Illuminate\Contracts\View\View;
use Maatwebsite\Excel\Concerns\FromQuery;
use Maatwebsite\Excel\Concerns\WithHeadings;
use Maatwebsite\Excel\Concerns\ShouldAutoSize;
use Maatwebsite\Excel\Concerns\WithMapping;
use Maatwebsite\Excel\Concerns\WithTitle;
use Maatwebsite\Excel\Events\AfterSheet;
use Maatwebsite\Excel\Concerns\WithEvents;
class AnomaliesExport implements FromQuery, WithTitle,WithHeadings,ShouldAutoSize,WithMapping,WithEvents{
use Exportable;
private $year;
public function __construct(int $year){
$this->year = $year;
}
public function map($anomalie): array{
return [
$anomalie->id,
$anomalie->updated_at,
$anomalie->titre,
$anomalie->type,
$anomalie->lot->produit->nom,
$anomalie->lot->caracteristiquep,
$anomalie->lot->quantite,
$anomalie->criticite,
$anomalie->test->nom,
$anomalie->diagnostique,
collect($anomalie->actions()->pluck('designation'))->implode('; '),
collect($anomalie->regles()->pluck('titre'))->implode('; '),
$anomalie->cause,
];
}
public function headings(): array{
return [
'Anomalie n°',
'Date',
'Titre',
'Source',
'Article',
'Caracteristique',
'Qté Retourné',
'Criticité (%)',
'Test réalisé',
'Problème',
'Actions Correctives',
'Règles Enfreintes',
'Cause racine',
];
}
public function query(){
return Anomalie::where('etat','=','traité')->whereYear('updated_at',$this->year)->orderBy('updated_at','desc');
}
public function title(): string{
return 'Journal des anomalies '.$this->year;
}
public function registerEvents(): array{
$styleArray = [
'borders' => [
'allBorders' => [
'borderStyle' => \PhpOffice\PhpSpreadsheet\Style\Border::BORDER_THIN,
],
],
];
return [
AfterSheet::class => function(AfterSheet $event) use($styleArray) {
$cpt = count(Anomalie::where('etat','=','traité')->whereYear('updated_at',$this->year)->get()) +1;
$cellRange = 'A1:M1';
$event->sheet->getStyle($cellRange)->ApplyFromArray($styleArray);
$event->sheet->getDelegate()->getStyle($cellRange)->getFont()->setName('Calibri');
$event->sheet->getDelegate()->getStyle($cellRange)->getFont()->setSize(14);
$event->sheet->getStyle('A2:A'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('B2:B'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('C2:C'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('D2:D'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('E2:E'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('F2:F'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('G2:G'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('H2:H'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('I2:I'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('J2:J'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('K2:K'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('L2:L'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('M2:M'.$cpt)->applyFromArray($styleArray);
},
];
}
}
<file_sep><?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Procede extends Model
{
public function atelier()
{
return $this->belongsTo('App\Models\Atelier');
}
public function produit()
{
return $this->belongsTo('App\Models\Produit');
}
public function audits()
{
return $this->hasMany('App\Models\Audit');
}
}
<file_sep><?php
namespace App\Http\Controllers\Gestionnaire;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
use App\Models\Regle;
use App\Models\User;
use App\Models\Produit;
use App\Models\Audit;
use Auth;
use App\Models\Anomalie;
class RegleController extends Controller
{
public function index(){
}
public function create(){
$action = route('regle.create');
$produits = Produit::all();
return view('quality.regle.form', compact('action','produits'));
}
public function store(Request $request){
$this->validate($request,[
'titre' => ['required'],
'produit' => 'required',
'contenu' => 'required'
]);
$regle = new Regle;
$regle->titre = $request->titre;
$regle->contenu = $request->contenu;
$regle->user_id = Auth::user()->id; // Id de l'utilisateur authentifié
if(!empty($request->id)){
$anomalie = Anomalie::findOrFail($request->id);
$regle->produit_id = $anomalie->lot->produit->id;
$regle->save();
return view('quality.anomalie.create', compact('anomalie'));
}
else{
if(!empty($request->idAudit) ){
$audit = Audit::findOrFail($request->idAudit);
$regle->produit_id = $request->produit;
$regle->save();
return view('quality.audit.create', compact('audit'));
}
else{
$regle->produit_id = $request->produit;
$regle->save();
return redirect(route('regle.list'))->with('successMsg',"Création d'une nouvelle règle qualité");
}
}
}
public function show()
{
$regles = Regle::paginate(10);
return view('quality.regle.list',compact('regles'));
}
public function edit(Regle $regle)
{
$action = route('regle.update', ['regle' => $regle]);
$produits = Produit::all();
return view('quality.regle.form',compact('regle','action','produits'));
}
public function update(Request $request, Regle $regle)
{
$this->validate($request,[
'titre' => 'required',
'produit' => 'required',
'contenu' => 'required'
]);
$regle->titre = $request->titre;
$regle->contenu = $request->contenu;
$regle->produit_id = $request->produit;
$regle->user_id = Auth::user()->id; // Id de l'utilisateur authentifié
$regle->save();
return redirect(route('regle.list'))->with('successMsg',"Modification de la règle qualité");
}
public function delete(Regle $regle)
{
$regle->delete();
return redirect(route('regle.list'));
}
}
<file_sep><?php
use Illuminate\Database\Seeder;
use Illuminate\Support\Facades\DB;
use Carbon\Carbon;
class UsersTableSeeder extends Seeder
{
/**
* Run the database seeds.
*
* @return void
*/
public function run()
{
DB::table('users')->insert([
'id' => '1',
'nom' => 'Ouared',
'prenom' => 'Nabil',
'numero_tel' => '+213550902696',
'service' => 'SI',
'email' => '<EMAIL>',
'password' => bcrypt('<PASSWORD>'),
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('users')->insert([
'id' => '2',
'nom' => 'Nacer',
'prenom' => 'Thinhinane',
'numero_tel' => '+213560208116',
'service' => 'Production',
'email' => '<EMAIL>',
'password' => bcrypt('<PASSWORD>'),
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('users')->insert([
'id' => '3',
'nom' => 'Bendjebbar',
'prenom' => 'Loubna',
'numero_tel' => '+213550902702',
'service' => 'Achat & Stock',
'email' => '<EMAIL>',
'password' => bcrypt('<PASSWORD>'),
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('users')->insert([
'id' => '4',
'nom' => 'Mimoune',
'prenom' => 'Fahima',
'numero_tel' => '+213550902702',
'service' => 'Commerciale',
'email' => '<EMAIL>',
'password' => bcrypt('<PASSWORD>'),
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('users')->insert([
'id' => '5',
'nom' => 'Mohamed',
'prenom' => 'Dzanouni',
'numero_tel' => '',
'service' => 'QHSE',
'email' => '<EMAIL>',
'password' => bcrypt('<PASSWORD>'),
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('users')->insert([
'id' => '6',
'nom' => 'Riche',
'prenom' => '<NAME>',
'numero_tel' => '',
'service' => 'Réparation',
'email' => '<EMAIL>',
'password' => bcrypt('<PASSWORD>'),
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('users')->insert([
'id' => '7',
'nom' => 'Hamraoui',
'prenom' => 'Yasmine',
'numero_tel' => '',
'service' => 'Réparation',
'email' => '<EMAIL>',
'password' => bcrypt('<PASSWORD>'),
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('users')->insert([
'id' => '8',
'nom' => 'Slimani',
'prenom' => 'Nadia',
'numero_tel' => '',
'service' => 'Réparation',
'email' => '<EMAIL>',
'password' => bcrypt('<PASSWORD>'),
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
}
}
<file_sep><?php
use Illuminate\Database\Seeder;
class DatabaseSeeder extends Seeder
{
/**
* Seed the application's database.
*
* @return void
*/
public function run()
{
$this->call(UsersTableSeeder::class);
$this->call(RolesTableSeeder::class);
$this->call(PermissionsTableSeeder::class);
$this->call(Model_Has_PermissionsTableSeeder::class);
$this->call(Role_Has_PermissionsTableSeeder::class);
$this->call(Model_Has_RolesTableSeeder::class);
$this->call(ProduitTableSeeder::class);
$this->call(CaracteristiqueTableSeeder::class);
$this->call(ActionTableSeeder::class);
$this->call(TestTableSeeder::class);
$this->call(ExamenTableSeeder::class);
$this->call(RegleTableSeeder::class);
$this->call(AtelierTableSeeder::class);
$this->call(AlertsTableSeeder::class);
$this->call(ProcedesTableSeeder::class);
$this->call(LotsTableSeeder::class);
$this->call(ClientTableSeeder::class);
$this->call(FournisseurTableSeeder::class);
}
}
<file_sep><?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Event extends Model
{
public function audit()
{
return $this->hasOne('App\Models\Audit');
}
public function inspection()
{
return $this->hasOne('App\Models\Inspection');
}
public function alert()
{
return $this->hasOne('App\Models\Alert');
}
public function user()
{
return $this->hasOne('App\User');
}
}
<file_sep><?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Attribut extends Model
{
public function anomalie()
{
return $this->belongsTo('App\Models\Anomalie');
}
public function audit()
{
return $this->belongsTo('App\Models\Audit');
}
public function inspection()
{
return $this->belongsTo('App\Models\Inspection');
}
public function action()
{
return $this->belongsTo('App\Models\Action');
}
}
<file_sep><?php
namespace App\Exports;
use App\Models\Inspection;
use Maatwebsite\Excel\Concerns\Exportable;
use Illuminate\Contracts\View\View;
use Maatwebsite\Excel\Concerns\FromQuery;
use Maatwebsite\Excel\Concerns\WithHeadings;
use Maatwebsite\Excel\Concerns\ShouldAutoSize;
use Maatwebsite\Excel\Concerns\WithMapping;
use Maatwebsite\Excel\Concerns\WithTitle;
use Maatwebsite\Excel\Events\AfterSheet;
use Maatwebsite\Excel\Concerns\WithEvents;
class InspectionsExport implements FromQuery, WithTitle,WithHeadings,ShouldAutoSize,WithMapping,WithEvents{
use Exportable;
// use RegistersEventListeners;
private $year;
public function __construct(int $year)
{
$this->year = $year;
}
public function map($inspection): array
{
return [
$inspection->updated_at,
$inspection->titre,
$inspection->lot->produit->nom,
$inspection->lot->caracteristiquep,
$inspection->lot->quantite,
($inspection->lot->quantite - $inspection->quantiteD),
$inspection->quantiteD,
number_format(($inspection->lot->quantite - $inspection->quantiteD)*100/$inspection->lot->quantite, 2, '.', ''),
number_format($inspection->quantiteD*100/($inspection->lot->quantite), 2, '.', ''),
$inspection->commentaire,
];
}
public function headings(): array
{
return [
'Date',
'Titre',
'Article',
'Caracteristique',
'Qté Testé',
'Bonne',
'Défectueuse',
'% Bonne',
'% Défectueuse',
'Observations',
];
}
public function query(){
return Inspection::where('etat','=','traité')->whereYear('updated_at',$this->year)->orderBy('updated_at','desc');
}
public function title(): string{
return 'Journal de qualité '.$this->year;
}
public function registerEvents(): array{
$styleArray = [
'borders' => [
'allBorders' => [
'borderStyle' => \PhpOffice\PhpSpreadsheet\Style\Border::BORDER_THIN,
//'color' => ['argb' => 'FFFF0000'],
],
],
];
return [
AfterSheet::class => function(AfterSheet $event) use($styleArray) {
$cpt = count(Inspection::where('etat','=','traité')->whereYear('updated_at',$this->year)->get()) +1;
$cellRange = 'A1:J1';
$event->sheet->getStyle($cellRange)->ApplyFromArray($styleArray);
$event->sheet->getDelegate()->getStyle($cellRange)->getFont()->setName('Calibri');
$event->sheet->getDelegate()->getStyle($cellRange)->getFont()->setSize(14);
$event->sheet->getStyle('A2:A'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('B2:B'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('C2:C'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('D2:D'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('E2:E'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('F2:F'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('G2:G'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('H2:H'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('I2:I'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('J2:J'.$cpt)->applyFromArray($styleArray);
},
];
}
}
<file_sep><?php
namespace App\Http\Controllers\Simple;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
use App\Models\Produit;
use App\Models\Procede;
use App\Models\Caracteristique;
use App\Exports\ProduitsExport;
use App\Imports\ProduitsImport;
use Maatwebsite\Excel\Facades\Excel;
class ProduitController extends Controller
{
/**
* @return \Illuminate\Support\Collection
*/
public function export(){
return Excel::download(new ProduitsExport, 'Articles.xlsx');
}
/**
* @return \Illuminate\Support\Collection
*/
public function import(){
Excel::import(new ProduitsImport,request()->file('file'));
return back();
}
public function index(){
return view('simple.produit.index');
}
public function create(){
$actionForm = route('produit.create');
return view('simple.produit.form', compact('actionForm'));
}
public function store(Request $request){
$this->validate($request, [
'nom' => 'required',
'modele' => 'required',
'prix' => 'integer',
'description' => 'required'
]);
$produit = new Produit;
$produit->nom = $request->nom;
$produit->type = $request->type;
$produit->modele = $request->modele;
$produit->prix = $request->prix;
$produit->description = $request->description;
$produit->save();
if(!empty($request->nomc)){
/* insert all caracteristics one by one */
for ($i=0; $i < count($request->nomc) ; $i++) {
$caracteristique = new Caracteristique;
$caracteristique->nom = $request->nomc[$i];
$caracteristique->produit_id = $produit->id;
$caracteristique->save();
}
}
if(!empty($request->designationp)){
/* insert all procede one by one */
for ($i=0; $i < count($request->designationp) ; $i++) {
$procede = new Procede;
$procede->designation = $request->designationp[$i];
$procede->description = $request->descriptionp[$i];
$procede->atelier_id = $request->atelierp[$i];
$procede->produit_id = $produit->id;
$procede->save();
}
}
return redirect(route('produit.list'))->with('successMsg',"L'article est ajouté avec succès");
}
public function edit(Produit $produit){
$actionForm = route('produit.update', ['produit' => $produit]);
return view('simple.produit.form',compact('produit','actionForm'));
}
public function update(Request $request, Produit $produit){
$this->validate($request,[
'nom' => 'required',
'modele' => 'required',
'type' => 'required',
'prix' => 'integer',
'description' => 'required'
]);
$produit->nom = $request->nom;
$produit->type = $request->type;
$produit->modele = $request->modele;
$produit->prix = $request->prix;
$produit->description = $request->description;
$produit->save();
Caracteristique::where('produit_id', $produit->id)->delete();
if(!empty($request->nomc)){
/* insert all caracteristics one by one */
for ($i=0; $i < count($request->nomc) ; $i++) {
$caracteristique = new Caracteristique;
$caracteristique->nom = $request->nomc[$i];
$caracteristique->produit_id = $produit->id;
$caracteristique->save();
}
}
Procede::where('produit_id', $produit->id)->delete();
if(!empty($request->designationp)){
/* insert all procede one by one */
for ($i=0; $i < count($request->designationp) ; $i++) {
$procede = new Procede;
$procede->designation = $request->designationp[$i];
$procede->description = $request->descriptionp[$i];
$procede->atelier_id = $request->atelierp[$i];
$procede->produit_id = $produit->id;
$procede->save();
}
}
return redirect(route('produit.list'))->with('successMsg',"L'article est modifié avec succès");
}
public function delete(Produit $produit){
$produit->delete();
return redirect(route('produit.list'));
}
}
<file_sep><?php
namespace App\Exports;
use App\Models\Audit;
use Maatwebsite\Excel\Concerns\Exportable;
use Illuminate\Contracts\View\View;
use Maatwebsite\Excel\Concerns\FromQuery;
use Maatwebsite\Excel\Concerns\WithHeadings;
use Maatwebsite\Excel\Concerns\ShouldAutoSize;
use Maatwebsite\Excel\Concerns\WithMapping;
use Maatwebsite\Excel\Concerns\WithTitle;
use Maatwebsite\Excel\Events\AfterSheet;
use Maatwebsite\Excel\Concerns\WithEvents;
class AuditsExport implements FromQuery, WithTitle,WithHeadings,ShouldAutoSize,WithMapping,WithEvents{
use Exportable;
private $year;
public function __construct(int $year){
$this->year = $year;
}
public function map($audit): array{
return [
$audit->id,
$audit->updated_at,
$audit->titre,
$audit->procede->designation,
$audit->procede->atelier->nom,
$audit->resultats,
collect($audit->actions()->pluck('designation'))->implode('; '),
collect($audit->regles()->pluck('titre'))->implode('; '),
$audit->commentaire,
];
}
public function headings(): array{
return [
'Audit n°',
'Date',
'Titre',
'Procédé métier',
'Atelier',
'Résultat',
'Actions Correctives',
'Règles enfreintes',
'Observations',
];
}
public function query(){
return Audit::where('etat','=','traité')->whereYear('updated_at',$this->year)->orderBy('updated_at','desc');
}
public function title(): string{
return 'Journal des audits '.$this->year;
}
public function registerEvents(): array{
$styleArray = [
'borders' => [
'allBorders' => [
'borderStyle' => \PhpOffice\PhpSpreadsheet\Style\Border::BORDER_THIN,
],
],
];
return [
AfterSheet::class => function(AfterSheet $event) use($styleArray) {
$cpt = count(Audit::where('etat','=','traité')->whereYear('updated_at',$this->year)->get()) +1;
$cellRange = 'A1:I1';
$event->sheet->getStyle($cellRange)->ApplyFromArray($styleArray);
$event->sheet->getDelegate()->getStyle($cellRange)->getFont()->setName('Calibri');
$event->sheet->getDelegate()->getStyle($cellRange)->getFont()->setSize(14);
$event->sheet->getStyle('A2:A'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('B2:B'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('C2:C'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('D2:D'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('E2:E'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('F2:F'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('G2:G'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('H2:H'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('I2:I'.$cpt)->applyFromArray($styleArray);
},
];
}
}
<file_sep><?php
namespace App\Imports;
use App\Models\Atelier;
use Maatwebsite\Excel\Concerns\ToModel;
use Maatwebsite\Excel\Concerns\WithHeadingRow;
class AteliersImport implements ToModel, WithHeadingRow
{
/**
* @param array $row
*
* @return \Illuminate\Database\Eloquent\Model|null
*/
public function model(array $row)
{
return new Atelier([
'nom' => $row['nom'],
'metier' => $row['metier'],
'description' => $row['description'],
]);
}
}
<file_sep><?php
namespace App\Exports;
use App\Models\Produit;
use Maatwebsite\Excel\Concerns\Exportable;
use Maatwebsite\Excel\Concerns\FromQuery;
use Maatwebsite\Excel\Concerns\WithHeadings;
use Maatwebsite\Excel\Concerns\ShouldAutoSize;
use Maatwebsite\Excel\Concerns\WithMapping;
use Maatwebsite\Excel\Concerns\WithTitle;
use Maatwebsite\Excel\Concerns\WithEvents;
use Maatwebsite\Excel\Events\AfterSheet;
class ProduitsExport implements FromQuery,WithMapping,WithHeadings,ShouldAutoSize,WithTitle,WithEvents
{
use Exportable;
public function map($article): array
{
return [
$article->id,
$article->nom,
$article->type,
$article->modele,
$article->description,
$article->prix,
collect($article->procedes()->pluck('designation'))->implode('; '),
collect($article->caracteristiques()->pluck('nom'))->implode('; '),
$article->created_at,
];
}
public function headings(): array
{
return [
'#',
'Nom',
'Type',
'Modèle',
'Description',
'Prix',
'Procedes',
'Caractéristique',
'Crée le',
];
}
public function title(): string
{
return 'Articles';
}
public function query()
{
return Produit::query();
}
public function registerEvents(): array{
$styleArray = [
'borders' => [
'allBorders' => [
'borderStyle' => \PhpOffice\PhpSpreadsheet\Style\Border::BORDER_THIN,
],
],
];
return [
AfterSheet::class => function(AfterSheet $event) use($styleArray) {
$cpt = count(Produit::all()) +1;
$cellRange = 'A1:I1';
$event->sheet->getStyle($cellRange)->ApplyFromArray($styleArray);
$event->sheet->getDelegate()->getStyle($cellRange)->getFont()->setName('Calibri');
$event->sheet->getDelegate()->getStyle($cellRange)->getFont()->setSize(14);
$event->sheet->getStyle('A2:A'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('B2:B'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('C2:C'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('D2:D'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('E2:E'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('F2:F'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('G2:G'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('H2:H'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('I2:I'.$cpt)->applyFromArray($styleArray);
},
];
}
}
<file_sep><?php
use Illuminate\Database\Seeder;
use Carbon\Carbon;
class ExamenTableSeeder extends Seeder
{
/**
* Run the database seeds.
*
* @return void
*/
public function run()
{
DB::table('examens')->insert([
'id' => '1',
'nom' => 'Vitesse de transfert des données en lecture',
'type' => 'Quantitatif',
'min' => '90',
'max' => '93',
'unite' => 'MB/s',
'test_id' => '1',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('examens')->insert([
'id' => '2',
'nom' => 'Vitesse de transfert des données en écriture',
'type' => 'Quantitatif',
'min' => '18',
'max' => '20',
'unite' => 'MB/s',
'test_id' => '1',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('examens')->insert([
'id' => '3',
'nom' => 'Capacité de stockage',
'type' => 'Quantitatif',
'min' => '15',
'max' => '17',
'unite' => 'Go',
'test_id' => '1',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('examens')->insert([
'id' => '4',
'nom' => 'Système de fichier FAT32',
'type' => 'Qualitatif',
'test_id' => '1',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('examens')->insert([
'id' => '5',
'nom' => 'Système de fichier NTFS',
'type' => 'Qualitatif',
'test_id' => '1',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('examens')->insert([
'id' => '6',
'nom' => 'Tension',
'type' => 'Quantitatif',
'min' => '249',
'max' => '251',
'unite' => 'Volt',
'test_id' => '2',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('examens')->insert([
'id' => '7',
'nom' => 'Courant',
'type' => 'Quantitatif',
'min' => '9',
'max' => '11',
'unite' => 'Ampére',
'test_id' => '2',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('examens')->insert([
'id' => '8',
'nom' => 'Puissance',
'type' => 'Quantitatif',
'min' => '2000',
'max' => '2010',
'unite' => 'Watt',
'test_id' => '2',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('examens')->insert([
'id' => '9',
'nom' => 'Résistance de température',
'type' => 'Quantitatif',
'min' => '20',
'max' => '85',
'unite' => 'Degré',
'test_id' => '2',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('examens')->insert([
'id' => '10',
'nom' => 'Longueur du câble',
'type' => 'Quantitatif',
'min' => '1',
'max' => '2',
'unite' => 'mètre',
'test_id' => '3',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('examens')->insert([
'id' => '11',
'nom' => 'Poids du câble',
'type' => 'Quantitatif',
'min' => '110',
'max' => '120',
'unite' => 'gramme',
'test_id' => '3',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('examens')->insert([
'id' => '12',
'nom' => 'Matériel isolation en PVC',
'type' => 'Qualitatif',
'test_id' => '3',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('examens')->insert([
'id' => '13',
'nom' => 'Trois boutons',
'type' => 'Qualitatif',
'test_id' => '4',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('examens')->insert([
'id' => '14',
'nom' => 'Lumière visible',
'type' => 'Qualitatif',
'test_id' => '4',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('examens')->insert([
'id' => '15',
'nom' => '<NAME>',
'type' => 'Qualitatif',
'test_id' => '4',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('examens')->insert([
'id' => '16',
'nom' => 'Résolution',
'type' => 'Quantitatif',
'min' => '1350',
'max' => '1450',
'unite' => 'Dpi',
'test_id' => '4',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('examens')->insert([
'id' => '17',
'nom' => 'Tension entrée',
'type' => 'Quantitatif',
'min' => '100',
'max' => '240',
'unite' => 'Volt',
'test_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('examens')->insert([
'id' => '18',
'nom' => '<NAME>',
'type' => 'Quantitatif',
'min' => '5',
'max' => '10',
'unite' => 'Volt',
'test_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('examens')->insert([
'id' => '19',
'nom' => '<NAME>',
'type' => 'Quantitatif',
'min' => '1',
'max' => '2',
'unite' => 'Ampère',
'test_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('examens')->insert([
'id' => '20',
'nom' => '<NAME>',
'type' => 'Quantitatif',
'min' => '1',
'max' => '2',
'unite' => 'Ampère',
'test_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('examens')->insert([
'id' => '21',
'nom' => 'Conductivité du cable',
'type' => 'Quantitatif',
'min' => '2',
'max' => '4',
'unite' => 'Ohms',
'test_id' => '6',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('examens')->insert([
'id' => '22',
'nom' => '<NAME> : connecteur USB',
'type' => 'Qualitatif',
'test_id' => '6',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('examens')->insert([
'id' => '23',
'nom' => '<NAME> : connecteur Micro USB',
'type' => 'Qualitatif',
'test_id' => '6',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('examens')->insert([
'id' => '24',
'nom' => 'Vitesse de transfert des données en lecture',
'type' => 'Quantitatif',
'min' => '88',
'max' => '90',
'unite' => 'MB/s',
'test_id' => '7',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('examens')->insert([
'id' => '25',
'nom' => 'Vitesse de transfert des données en écriture',
'type' => 'Quantitatif',
'min' => '18',
'max' => '20',
'unite' => 'MB/s',
'test_id' => '7',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('examens')->insert([
'id' => '26',
'nom' => 'Capacité de stockage',
'type' => 'Quantitatif',
'min' => '31',
'max' => '33',
'unite' => 'Go',
'test_id' => '7',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('examens')->insert([
'id' => '27',
'nom' => 'Système de fichier FAT32',
'type' => 'Qualitatif',
'test_id' => '7',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('examens')->insert([
'id' => '28',
'nom' => 'Système de fichier NTFS',
'type' => 'Qualitatif',
'test_id' => '7',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
}
}
<file_sep><?php
namespace App\Http\Controllers\Gestionnaire;
use DB;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
use App\Models\Lot;
use App\Models\Test;
use App\Models\Inspection;
use App\Models\Alert;
use App\Models\Produit;
use App\Models\Examen;
use App\Models\Reponse;
use App\Models\Event;
use Auth;
use Carbon\Carbon;
use Redirect,Response;
use PDF;
use App\Exports\InspectionsExport;
use Maatwebsite\Excel\Facades\Excel;
class InspectionController extends Controller{
public function export(){
$year = Carbon::now()->format('Y');
return (new InspectionsExport($year))->download('Journal des inspections '.$year.'.xlsx');
}
public function index(){
$inspections = Inspection::orderBy('id','desc')->paginate(10);
return view('quality.inspection.index',compact('inspections'));
}
public function create(Request $request){
$produits = Produit::where('type', '=' , 'Fini' )->get();
return view('quality.inspection.create',compact('produits'));
}
public function createFrom(Request $request, $id){
$event = Event::findOrFail($id);
$event->rappel = "false"; // update rappel variable
$event->save();
$inspection = Inspection::where('id', '=' , $event->inspection_id)->first();
if($event->alert != null){
$event->alert->etat ="en cours";
$event->alert->save();
}
$produits = Produit::where('type', '=' , 'Fini' )->get();
return view('quality.inspection.create', compact('inspection','produits'));
}
public function postCreateStep1(Request $request){
$request->validate([
'titre' => 'required',
'date' => 'required',
'description' => 'required',
'productimg' => 'image|mimes:jpeg,png,jpg,gif,svg',
'produit' => 'required',
'caracteristiquep' => 'required',
'quantite' => ['required','integer'],
'test' => 'required'
]);
if(empty($request->id)){
$inspection = new Inspection();
}
else{
$inspection = Inspection::findOrFail($request->id);
}
if(empty($inspection->lot)){
$lot = new Lot();
}
else{
$lot = Lot::findOrFail($inspection->lot_id);
}
$lot->quantite = $request->quantite;
$lot->produit_id = $request->produit;
$lot->caracteristiquep = $request->caracteristiquep;
$lot->save();
$inspection->lot_id = $lot->id;
$inspection->titre = $request->titre;
$inspection->description = $request->description;
$inspection->test_id = $request->test;
$inspection->user_id = Auth::user()->id; // Id de l'utilisateur authentifie
if(!empty($request->productimg)){
$fileName = "lot-" . time() . '.' . request()->productimg->getClientOriginalExtension();
$request->productimg->storeAs('lotImage', $fileName);
$inspection->productimg = $fileName;
} else{
$inspection->productImg = null;
}
$inspection->etat="nouveau";
$inspection->step = 2;
$test_id = $inspection->test_id;
$inspection->save();
if(empty($request->id)){
$event = new Event();
$event->title = $inspection->titre;
$event->start = $request->date;
$event->end = $request->date;
$event->type = 'Inspection';
$event->inspection_id = $inspection->id;
$event->save();
}
else{
$update_event = DB::table('events')
->where('inspection_id', $inspection->id )
->update(['title' => $inspection->titre, 'start' => $request->date ,
'end' => $request->date]);
}
$examens = Examen::where('test_id', '=' , $test_id )->get();
$produits = Produit::where('type', '=' , 'Fini' )->get();
return view('quality.inspection.create',compact('produits','inspection','examens'));
}
public function postCreateStep2(Request $request){
$inspection = Inspection::findOrFail($request->id);
$result = "Les réponses des examens sont toutes correctes";
if (!empty($request->ReponsesEtat)){
for ($i=0; $i < count($request->ReponsesEtat) ; $i++) {
if ($request->ReponsesEtat[$i] =="Incorrect"){
$result = "Les réponses des examens ne sont pas toutes correctes";
}
DB::table('reponses')
->updateOrInsert(
['inspection_id' => $inspection->id, 'examen_id' => $request->ExamensIdd[$i]],
['valeur' => $request->ReponsesValeur[$i] ,
'examen_id' => $request->ExamensIdd[$i] ,
'inspection_id' => $inspection->id,
'etat' => $request->ReponsesEtat[$i] ]
);
}
}
$test_id = $inspection->test_id;
$inspection->etat="en cours";
$inspection->step = 3;
$inspection->resultats = $result;
$inspection->save();
$examens = Examen::where('test_id', '=' , $test_id )->get();
$produits = Produit::where('type', '=' , 'Fini' )->get();
return view('quality.inspection.create',compact('produits','inspection','examens'));
}
public function store(Request $request){
$request->validate([
'quantiteD' => 'integer',
]);
$inspection = Inspection::findOrFail($request->id);
if (!empty($request->commentaire)){
$inspection->commentaire = $request->commentaire;
}
if (!empty($request->quantiteD)){
$inspection->quantiteD = $request->quantiteD;
}
else{
$inspection->quantiteD = 0;
}
$inspection->etat="traité";
$inspection->step = 1;
$inspection->save();
if ($request->anomalie){
$lotA = DB::table('lots')->insertGetId([
'quantite' => $request->quantiteD,
'caracteristiquep' => $inspection->lot->caracteristiquep,
'produit_id' => $inspection->lot->produit->id
]);
/* automaticly create an associated anomaly */
$anomalie_id = DB::table('anomalies')->insertGetId([
'lot_id' => $lotA,
'atelier_id' => 5, // atelier de controle dans le seeders
'test_id' => $inspection->test_id,
'type' => 'Retour production',
'step' => 3,
'etat' => "en cours",
'titre' => $inspection->titre. ' - De Inspection',
'description' => $inspection->description,
'resultats' => $inspection->resultats,
'created_at' => Carbon::now(),
'updated_at' => Carbon::now(),
]);
/* Associate an alert to anomaly */
$alert = new Alert;
$alert->type = 'Retour production';
$alert->description = $inspection->description;
$alert->atelier_id = 5; // atelier de controle dans le seeders
$alert->sent = 1;
$alert->anomalie_id = $anomalie_id;
$alert->etat = "en cours";
$alert->lot_id = $lotA;
$alert->save();
$reponses = Reponse::where('inspection_id', '=' , $inspection->id )->get();
for ($i=0; $i < count($reponses) ; $i++) {
$reponses[$i]->anomalie_id= $anomalie_id;
$reponses[$i]->save();
}
$inspection->anomalie_id = $anomalie_id;
$inspection->save();
return redirect(route('anomalie.createFrom',$anomalie_id));
}
return redirect(route('inspection.dashbord'))->with('successMsg',"Création d'une nouvelle inspection");
}
public function delete(Inspection $inspection){
$inspection->delete();
return redirect(route('inspection.dashbord'));
}
public function previous(Inspection $inspection){
$inspection->step -= 1;
if($inspection->step < 1) $inspection->step=1;
$inspection->save();
$produits = Produit::where('type', '=' , 'Fini' )->get();
$test_id = $inspection->test_id;
$examens = Examen::where('test_id', '=' , $test_id )->get();
return view('quality.inspection.create', compact('inspection','produits','examens'));
}
public function edit(Inspection $inspection){
$test_id = $inspection->test_id;
$inspection->step = 1;
$inspection->etat = "en cours";
$examens = Examen::where('test_id', '=' , $test_id )->get();
$produits = Produit::where('type', '=' , 'Fini' )->get();
return view('quality.inspection.create',compact('produits','inspection','examens'));
}
function generate_pdf(Inspection $inspection) {
$pdf = PDF::loadView('pdf.ficheInspection', compact('inspection'));
return $pdf->stream('Fiche.pdf');
}
public function view(Inspection $inspection){
return view('quality.inspection.view',compact('inspection'));
}
}
<file_sep><?php
namespace App\Http\Controllers\Gestionnaire;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
use App\Models\Examen;
use App\Models\Test;
class TestController extends Controller
{
public function index(){
}
public function create(Request $request){
$action = route('test.create');
return view('quality.test.form', compact('action'));
}
public function store(Request $request){
$this->validate($request,[
'description' => 'required',
'nom' => 'required',
'type' => 'required'
]);
$test = new Test;
$test->type = $request->type;
$test->nom = $request->nom;
$test->description = $request->description;
$test->save();
if (!empty($request->nomE))
{
/* insert all exams one by one */
for ($i=0; $i < count($request->nomE) ; $i++) {
$examen = new Examen;
$examen->type = $request->typeE[$i];
$examen->nom = $request->nomE[$i];
$examen->test_id = $test->id; // after test is inserted u can access his id
if ($examen->type == "Quantitatif"){
$examen->min = $request->min[$i];
$examen->max = $request->max[$i];
$examen->unite = $request->unite[$i];
}
/* if ($examen->type == "Qualitatif"){
$examen->reponse1 = $request->reponse[$i];
$examen->reponse2 = $request->reponseTwo[$i];
}*/
$examen->save();
}
}
return redirect()->route('test.list')->with('successMsg',"Création d'un nouveau test");
}
public function show(){
$tests = Test::paginate(10);
return view('quality.test.list',compact('tests'));
}
public function edit(Test $test){
$action = route('test.update', ['test' => $test]);
return view('quality.test.form',compact('test','action'));
}
public function update(Request $request, Test $test){
$this->validate($request,[
'description' => 'required',
'nom' => 'required',
'type' => 'required'
]);
$test->type = $request->type;
$test->nom = $request->nom;
$test->description = $request->description;
$test->save();
Examen::where('test_id', $test->id)->delete();
/* insert all exams one by one */
if (!empty($request->nomE)){
for ($i=0; $i < count($request->nomE) ; $i++) {
$examen = new Examen;
$examen->type = $request->typeE[$i];
$examen->nom = $request->nomE[$i];
$examen->test_id = $test->id; // after test is inserted u can access his id
if ($examen->type == "Quantitatif"){
$examen->min = $request->min[$i];
$examen->max = $request->max[$i];
$examen->unite = $request->unite[$i];
}
/* if ($examen->type == "Qualitatif"){
$examen->question = $request->question[$i];
//$examen->reponse2 = $request->reponseTwo[$i];
} */
$examen->save();
}
}
return redirect(route('test.list'))->with('successMsg',"Modification du test");
}
public function delete(Test $test){
$test->delete();
return redirect(route('test.list'));
}
public function storeExamen(Request $request){
$type = $request->typeE;
if ( $type == "Quantitatif"){
$this->validate($request,[
'min' => 'required',
'max' => 'required',
'unite' => 'required'
]);
$examen = new Examen;
$examen->type = $request->typeE;
$examen->nom = $request->nomE;
$examen->min = $request->min;
$examen->max = $request->max;
$examen->unite = $request->unite;
$examen->test_id = $test_id;
$examen->save();
return redirect(route('test.create'));
}
if ( $type == "Qualitatif")
{
$examen = new Examen;
$examen->type = $request->typeE;
$examen->nom = $request->nomE;
$examen->reponse = $request->reponse;
$examen->test_id = $test_id;
$examen->save();
return redirect(route('test.create'));
}
}
}
<file_sep><?php
namespace App\Exports;
use App\Models\Fournisseur;
use Maatwebsite\Excel\Concerns\Exportable;
use Maatwebsite\Excel\Concerns\FromQuery;
use Maatwebsite\Excel\Concerns\WithHeadings;
use Maatwebsite\Excel\Concerns\ShouldAutoSize;
use Maatwebsite\Excel\Concerns\WithMapping;
use Maatwebsite\Excel\Concerns\WithTitle;
use Maatwebsite\Excel\Concerns\WithEvents;
use Maatwebsite\Excel\Events\AfterSheet;
class FournisseursExport implements FromQuery,WithMapping,WithHeadings,ShouldAutoSize,WithTitle,WithEvents
{
use Exportable;
public function map($fournisseur): array
{
return [
$fournisseur->id,
$fournisseur->nom,
$fournisseur->adresse,
$fournisseur->description,
$fournisseur->created_at,
];
}
public function headings(): array
{
return [
'#',
'Nom',
'Adresse',
'Description',
'Créé le',
];
}
public function title(): string
{
return 'Fournisseurs';
}
public function query()
{
return Fournisseur::query();
}
public function registerEvents(): array{
$styleArray = [
'borders' => [
'allBorders' => [
'borderStyle' => \PhpOffice\PhpSpreadsheet\Style\Border::BORDER_THIN,
],
],
];
return [
AfterSheet::class => function(AfterSheet $event) use($styleArray) {
$cpt = count(Fournisseur::all()) +1;
$cellRange = 'A1:E1';
$event->sheet->getStyle($cellRange)->ApplyFromArray($styleArray);
$event->sheet->getDelegate()->getStyle($cellRange)->getFont()->setName('Calibri');
$event->sheet->getDelegate()->getStyle($cellRange)->getFont()->setSize(14);
$event->sheet->getStyle('A2:A'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('B2:B'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('C2:C'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('D2:D'.$cpt)->applyFromArray($styleArray);
$event->sheet->getStyle('E2:E'.$cpt)->applyFromArray($styleArray);
},
];
}
}
<file_sep><?php
use Illuminate\Database\Seeder;
use Carbon\Carbon;
class ProcedesTableSeeder extends Seeder
{
/**
* Run the database seeds.
*
* @return void
*/
public function run()
{
DB::table('procedes')->insert([
'id' => '1',
'designation' => 'Programmation des machines SMT',
'description' => '',
'atelier_id' => '1',
'produit_id' => '7',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('procedes')->insert([
'id' => '2',
'designation' => 'Programmation des cartes électroniques',
'description' => '',
'atelier_id' => '1',
'produit_id' => '7',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('procedes')->insert([
'id' => '3',
'designation' => 'Sertissage câble alimentation',
'description' => '',
'atelier_id' => '3',
'produit_id' => '6',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('procedes')->insert([
'id' => '4',
'designation' => 'Soudage câble alimentation',
'description' => '',
'atelier_id' => '3',
'produit_id' => '6',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('procedes')->insert([
'id' => '5',
'designation' => 'Emballage câble alimentation',
'description' => '',
'atelier_id' => '3',
'produit_id' => '6',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('procedes')->insert([
'id' => '6',
'designation' => 'Injection plastique FD',
'description' => '',
'atelier_id' => '3',
'produit_id' => '6',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('procedes')->insert([
'id' => '7',
'designation' => 'Injection plastique souris',
'description' => '',
'atelier_id' => '2',
'produit_id' => '3',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('procedes')->insert([
'id' => '8',
'designation' => 'Injection plastique chargeur',
'description' => '',
'atelier_id' => '2',
'produit_id' => '4',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('procedes')->insert([
'id' => '9',
'designation' => 'Soudage connecteurs câble USB',
'description' => '',
'atelier_id' => '2',
'produit_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('procedes')->insert([
'id' => '10',
'designation' => 'Injection PVC câble USB',
'description' => '',
'atelier_id' => '2',
'produit_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('procedes')->insert([
'id' => '11',
'designation' => 'Emballage câble USB',
'description' => '',
'atelier_id' => '2',
'produit_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('procedes')->insert([
'id' => '12',
'designation' => 'Montage souris',
'description' => '',
'atelier_id' => '4',
'produit_id' => '3',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('procedes')->insert([
'id' => '13',
'designation' => 'Emballage souris',
'description' => '',
'atelier_id' => '4',
'produit_id' => '3',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('procedes')->insert([
'id' => '14',
'designation' => 'Montage chargeur',
'description' => '',
'atelier_id' => '4',
'produit_id' => '4',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('procedes')->insert([
'id' => '15',
'designation' => 'Emballage chargeur',
'description' => '',
'atelier_id' => '4',
'produit_id' => '4',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('procedes')->insert([
'id' => '16',
'designation' => 'Montage FD',
'description' => '',
'atelier_id' => '4',
'produit_id' => '1',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('procedes')->insert([
'id' => '17',
'designation' => 'Emballage FD',
'description' => '',
'atelier_id' => '4',
'produit_id' => '1',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('procedes')->insert([
'id' => '18',
'designation' => 'Programmation FD',
'description' => '',
'atelier_id' => '5',
'produit_id' => '1',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('procedes')->insert([
'id' => '19',
'designation' => 'Emballage RAM',
'description' => '',
'atelier_id' => '5',
'produit_id' => '7',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
}
}
<file_sep><?php
namespace App\Http\Controllers\Simple;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
use App\Models\Atelier;
use App\Exports\AteliersExport;
use App\Imports\AteliersImport;
use Maatwebsite\Excel\Facades\Excel;
class AtelierController extends Controller
{
public function export(){
return Excel::download(new AteliersExport(), 'Ateliers.xlsx');
}
public function import(){
Excel::import(new AteliersImport,request()->file('file'));
return back();
}
public function index(){
$ateliers = Atelier::paginate(10);
return view('simple.atelier.index',compact('ateliers'));
}
public function create(){
$actionForm = route('atelier.create');
return view('simple.atelier.form', compact('actionForm'));
}
public function store(Request $request){
$this->validate($request,[
'description' => 'required',
'nom' => 'required',
'metier' => 'required'
]);
$atelier = new Atelier;
$atelier->nom = $request->nom;
$atelier->metier = $request->metier;
$atelier->description = $request->description;
$atelier->save();
return redirect(route('atelier.list'))->with('successMsg',"L'atelier est ajouté avec succès");
}
public function edit(Atelier $atelier){
$actionForm = route('atelier.update', ['atelier' => $atelier]);
return view('simple.atelier.form',compact('atelier','actionForm'));
}
public function update(Request $request, Atelier $atelier){
$this->validate($request,[
'description' => 'required',
'nom' => 'required',
'metier' => 'required'
]);
$atelier->nom = $request->nom;
$atelier->metier = $request->metier;
$atelier->description = $request->description;
$atelier->save();
return redirect(route('atelier.list'))->with('successMsg',"L'atelier est modifié avec succès");
}
public function delete(Atelier $atelier){
$atelier->delete();
return redirect(route('atelier.list'));
}
}
<file_sep><?php
use Illuminate\Database\Seeder;
use Carbon\Carbon;
class FournisseurTableSeeder extends Seeder
{
/**
* Run the database seeds.
*
* @return void
*/
public function run()
{
DB::table('fournisseurs')->insert([
'id' => '1',
'nom' => '<NAME>',
'description' => '',
'adresse' => 'Rue Sadani M<NAME>-Blida',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('fournisseurs')->insert([
'id' => '2',
'nom' => 'Aitcom Pub',
'description' => '',
'adresse' => 'place 11 mars, villa N°16 plan de partition N°42, Annaba',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('fournisseurs')->insert([
'id' => '3',
'nom' => '<NAME>',
'description' => '',
'adresse' => '89, Lot Vincent II Bouzareah, Alger',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('fournisseurs')->insert([
'id' => '4',
'nom' => 'Bouhoun Pub',
'description' => '',
'adresse' => 'Coopérative el nahda - n 41 Bir Khadem, Alger',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('fournisseurs')->insert([
'id' => '5',
'nom' => 'DATOTEK',
'description' => '',
'adresse' => 'Cité meriem N°07 "A", Baraki Alger',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('fournisseurs')->insert([
'id' => '6',
'nom' => '<NAME>',
'description' => '',
'adresse' => 'Bou Ismail, Tipaza',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('fournisseurs')->insert([
'id' => '7',
'nom' => '<NAME>',
'description' => '',
'adresse' => 'Zone activité Zouine Aaba Ali Birtouta -alger',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('fournisseurs')->insert([
'id' => '8',
'nom' => '<NAME>',
'description' => '',
'adresse' => 'Succursale N° 26 lot eldjanah lakhdar garidi Kouba,Alger',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('fournisseurs')->insert([
'id' => '9',
'nom' => '<NAME>',
'description' => '',
'adresse' => 'Ain Roumana,Blida',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('fournisseurs')->insert([
'id' => '10',
'nom' => '<NAME>',
'description' => '',
'adresse' => 'Cheraga,Alger',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('fournisseurs')->insert([
'id' => '11',
'nom' => '<NAME>',
'description' => '',
'adresse' => 'Boulevard du 11 Décembre 1960 ,Lot 65, El Biar',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('fournisseurs')->insert([
'id' => '12',
'nom' => '<NAME>',
'description' => '',
'adresse' => 'Les eucalyptus,Alger',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('fournisseurs')->insert([
'id' => '13',
'nom' => '<NAME>',
'description' => '',
'adresse' => 'Baraki,Alger',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
}
}
<file_sep><?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Anomalie extends Model
{
protected $fillable = [
'titre','description','diagnostique','cause','type'
];
public function regles()
{
return $this->belongsToMany('App\Models\Regle');
}
public function charges()
{
return $this->hasMany('App\Models\Charge');
}
public function inspections()
{
return $this->hasMany('App\Models\Inspection');
}
public function fichiers()
{
return $this->hasMany('App\Models\Fichier');
}
public function attributs()
{
return $this->hasMany('App\Models\Attribut');
}
public function reparateur()
{
return $this->belongsTo('App\User');
}
public function test()
{
return $this->belongsTo('App\Models\Test');
}
public function lot()
{
return $this->belongsTo('App\Models\Lot');
}
public function atelier()
{
return $this->belongsTo('App\Models\Atelier');
}
public function client()
{
return $this->belongsTo('App\Models\Client');
}
public function fournisseur()
{
return $this->belongsTo('App\Models\Fournisseur');
}
public function actions()
{
return $this->belongsToMany('App\Models\Action');
}
}
<file_sep><?php
use Illuminate\Database\Seeder;
class RolesTableSeeder extends Seeder
{
/**
* Run the database seeds.
*
* @return void
*/
public function run()
{
DB::table('roles')->insert([
'name' => 'admin',
'guard_name' => 'web',
'description' => 'gestion du site entier + privileges utilisateurs (configuration)',
]);
DB::table('roles')->insert([
'name' => 'gestionnaire',
'guard_name' => 'web',
'description' => 'gestion du controle et suivi de la qualité des produits',
]);
DB::table('roles')->insert([
'name' => 'simple',
'guard_name' => 'web',
'description' => 'lanceur d"alerte anomalie produit',
]);
}
}
<file_sep><?php
use Illuminate\Database\Seeder;
use Carbon\Carbon;
class CaracteristiqueTableSeeder extends Seeder
{
/**
* Run the database seeds.
*
* @return void
*/
public function run()
{
DB::table('caracteristiques')->insert([
'nom' => '4 Go',
'produit_id' => '1',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => '8 Go',
'produit_id' => '1',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => '16 Go',
'produit_id' => '1',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => '32 Go',
'produit_id' => '1',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => '64 Go',
'produit_id' => '1',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => '16 Go',
'produit_id' => '2',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => '32 Go',
'produit_id' => '2',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => '64 Go',
'produit_id' => '2',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => '128 Go',
'produit_id' => '2',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => '256 Go',
'produit_id' => '2',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'Sans fils',
'produit_id' => '3',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'Filaire',
'produit_id' => '3',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'Rapide adaptative',
'produit_id' => '4',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'Type C',
'produit_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'Luna',
'produit_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'Iphone',
'produit_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'PC',
'produit_id' => '6',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'LAPTOP',
'produit_id' => '6',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'Onduleur',
'produit_id' => '6',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => '2 GB',
'produit_id' => '7',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => '4 GB',
'produit_id' => '7',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => '8 GB',
'produit_id' => '7',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'Rouge',
'produit_id' => '8',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'Vert',
'produit_id' => '8',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'Bleu',
'produit_id' => '8',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'Male',
'produit_id' => '9',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'Female',
'produit_id' => '9',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => '1 m',
'produit_id' => '10',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => '2 m',
'produit_id' => '10',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => '3 m',
'produit_id' => '10',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'Rouge',
'produit_id' => '11',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'Vert',
'produit_id' => '11',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'Bleu',
'produit_id' => '11',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'USB 2.0',
'produit_id' => '12',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'USB 3.0',
'produit_id' => '12',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'RAM DDR',
'produit_id' => '12',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'Micro A',
'produit_id' => '13',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'Micro C',
'produit_id' => '13',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'Micro iPhone',
'produit_id' => '13',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'USB A',
'produit_id' => '13',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'Rouge',
'produit_id' => '14',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'Vert',
'produit_id' => '14',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'Bleu',
'produit_id' => '14',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'Connecteur USB',
'produit_id' => '15',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'Connecteur Micro USB',
'produit_id' => '15',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'Connecteur Type C',
'produit_id' => '15',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'Connecteur Iphone',
'produit_id' => '15',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'PC',
'produit_id' => '16',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'Treflle',
'produit_id' => '16',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'Onduleur',
'produit_id' => '16',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('caracteristiques')->insert([
'nom' => 'Catel',
'produit_id' => '16',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
}
}
<file_sep><?php
namespace App\Http\Controllers\Admin;
use App\User;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Hash;
use App\Http\Controllers\Controller;
use Spatie\Permission\Models\Role;
use Spatie\Permission\Models\Permission;
class UserController extends Controller
{
public function index(){
$users = User::all();
return view('admin.user.index',compact('users'));
}
public function create(){
$roles = Role::all();
$permissions = Permission::all();
$action = route('user.create');
return view('admin.user.form',compact('roles','permissions','action'));
}
public function store(Request $request){
$this->validate($request,[
'nom' => ['required', 'string', 'max:255'],
'prenom' => ['required', 'string', 'max:255'],
'numero_tel' => ['required', 'string', 'max:255'],
'service' => ['required', 'string', 'max:255'],
'email' => ['required', 'string', 'email', 'max:255', 'unique:users'],
'password' => ['<PASSWORD>', 'string', 'min:8'],
]);
$user = User::create($request->all());
$user->password = <PASSWORD>::make($request->password);
$user->syncRoles($request->roles);
$user->syncPermissions($request->permissions);
$user->save();
return redirect(route('user.dashbord'))->with('successMsg','Utilisateur créé avec succès');
}
public function edit(User $user){
$action = route('user.update', ['user' => $user]);
$roles = Role::all();
$permissions = Permission::all();
return view('admin.user.form',compact('user','roles','permissions','action'));
}
public function update(Request $request,User $user){
$user->nom = $request->nom;
$user->prenom = $request->prenom;
$user->email = $request->email;
$user->numero_tel = $request->numero_tel;
$user->service = $request->service;
$user->syncRoles($request->roles);
$user->syncPermissions($request->permissions);
$user->save();
return redirect(route('user.dashbord'))
->with('successMsg','Utilisateur modifié avec succès');
}
public function delete(User $user){
$user->delete();
return redirect(route('user.dashbord'));
}
}
<file_sep><?php
use Illuminate\Database\Seeder;
use Carbon\Carbon;
class RegleTableSeeder extends Seeder
{
/**
* Run the database seeds.
*
* @return void
*/
public function run()
{
DB::table('regles')->insert([
'id' => '1',
'titre' => 'Compatibilité',
'contenu' => 'toutes les versions de Windows, Mac Os version 10.4 ou version ultérieure, Linux Kernel 2.6 ou version ultérieure',
'produit_id' => '3',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '2',
'titre' => 'Protocole USB',
'contenu' => 'USB 2.0',
'produit_id' => '3',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '3',
'titre' => 'Résolution',
'contenu' => '1400 Dpi',
'produit_id' => '3',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '4',
'titre' => 'Nombre de boutons',
'contenu' => '3',
'produit_id' => '3',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '5',
'titre' => 'Capteur',
'contenu' => 'Type : optique, Lumiére visible : Oui, Couleur de la lumière : rouge',
'produit_id' => '3',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '6',
'titre' => 'Longeur cable',
'contenu' => '1,3 métres',
'produit_id' => '3',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '7',
'titre' => 'Molette',
'contenu' => 'Ordinaire',
'produit_id' => '3',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '8',
'titre' => 'Dimensions',
'contenu' => '67 mm x 117 mm x 35 mm',
'produit_id' => '3',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '9',
'titre' => 'Poids',
'contenu' => '85 grs',
'produit_id' => '3',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '10',
'titre' => 'Type de connexion',
'contenu' => 'Connectivité avancée sans fils 2,4 GHz',
'produit_id' => '3',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '11',
'titre' => 'Interface de connexion',
'contenu' => 'Récepteur USB',
'produit_id' => '3',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '12',
'titre' => 'Distance de travail',
'contenu' => '10 Métres',
'produit_id' => '3',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '13',
'titre' => 'Compatibilité',
'contenu' => 'Toutes les appareils avec un cable USB',
'produit_id' => '4',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '14',
'titre' => 'Dimensions',
'contenu' => '8 cm x 3,8 cm x 2,5 cm',
'produit_id' => '4',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '15',
'titre' => 'Poids',
'contenu' => '20 grs',
'produit_id' => '4',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '16',
'titre' => 'Puissance',
'contenu' => 'Sortie : 5V, 2.1A et Entrée : 100-240V , 0.5A',
'produit_id' => '4',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '17',
'titre' => 'Compatibilité',
'contenu' => 'toutes les versions de Windows, Mac Os version 10.4 ou version ultérieure, Linux Kernel 2.6 ou version ultérieure',
'produit_id' => '1',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '18',
'titre' => 'Protocole USB',
'contenu' => 'USB 2.0',
'produit_id' => '1',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '19',
'titre' => 'Connectivité',
'contenu' => '1x haute vitesse, 4pin USB,type A',
'produit_id' => '1',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '20',
'titre' => 'Branchement',
'contenu' => 'plug and play',
'produit_id' => '1',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '21',
'titre' => 'Températeure',
'contenu' => 'Min : 0° , Max : 60°',
'produit_id' => '1',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '22',
'titre' => 'Dimensions',
'contenu' => '67mm x 117mm x 35mm',
'produit_id' => '1',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '23',
'titre' => 'Poids',
'contenu' => '85 grs',
'produit_id' => '1',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '24',
'titre' => 'Compatibilité',
'contenu' => 'toutes les versions de Windows, Mac Os version 10.4 ou version ultérieure, Linux Kernel 2.6 ou version ultérieure',
'produit_id' => '2',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '25',
'titre' => 'Protocole USB',
'contenu' => 'USB 3.0',
'produit_id' => '2',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '26',
'titre' => 'Branchement',
'contenu' => 'plug and play',
'produit_id' => '2',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '27',
'titre' => 'Températeure',
'contenu' => 'Min : 0° , Max : 60°',
'produit_id' => '2',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '28',
'titre' => 'Tension',
'contenu' => '250 V , Courant 10 A',
'produit_id' => '6',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '29',
'titre' => 'Compatibilité',
'contenu' => 'Cable alimentation Eur Plug adapté aux moniteurs,ordinateurs,téleviseurs,imprimantes, scanners et projecteurs, code machine , brouilloire, cuiseur à riz',
'produit_id' => '6',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '30',
'titre' => 'Résistance de températeure',
'contenu' => 'Max : 65°',
'produit_id' => '6',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '31',
'titre' => 'Puissance nominale',
'contenu' => '2000 Watt',
'produit_id' => '6',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '32',
'titre' => 'Matériaux',
'contenu' => 'Ignifuge en PVC, noyeau de cuivre de haute qualité',
'produit_id' => '6',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '33',
'titre' => 'Longueur du cable',
'contenu' => '1,2 métres',
'produit_id' => '6',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '34',
'titre' => 'Poids',
'contenu' => '115 grs',
'produit_id' => '6',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '35',
'titre' => 'Type de charge',
'contenu' => 'Universel',
'produit_id' => '5',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '36',
'titre' => 'Entrées',
'contenu' => 'Entrée A : USB, Entrée B : Micro USB ',
'produit_id' => '5',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '37',
'titre' => 'Transfert de données mobiles',
'contenu' => 'Oui',
'produit_id' => '5',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('regles')->insert([
'id' => '38',
'titre' => 'Longueur du câble',
'contenu' => '1 mètre',
'produit_id' => '5',
'user_id' => '5',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
}
}
<file_sep><?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Audit extends Model
{
public function user()
{
return $this->belongsTo('App\User');
}
public function procede()
{
return $this->belongsTo('App\Models\Procede');
}
public function atelier()
{
return $this->belongsTo('App\Models\Atelier');
}
public function attributs()
{
return $this->hasMany('App\Models\Attribut');
}
public function actions()
{
return $this->belongsToMany('App\Models\Action');
}
public function event()
{
return $this->belongsTo('App\Models\Event');
}
public function questionnaires()
{
return $this->hasMany('App\Models\Questionnaire');
}
public function regles()
{
return $this->belongsToMany('App\Models\Regle');
}
}
<file_sep><?php
namespace App\Http\Controllers\Simple;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
use App\Models\Client;
use App\Exports\ClientsExport;
use App\Imports\ClientsImport;
use Maatwebsite\Excel\Facades\Excel;
class ClientController extends Controller
{
/**
* @return \Illuminate\Support\Collection
*/
public function export(){
return Excel::download(new ClientsExport, 'clients.xlsx');
}
/**
* @return \Illuminate\Support\Collection
*/
public function import(){
Excel::import(new ClientsImport,request()->file('file'));
return back();
}
public function index(){
$clients = client::paginate(15);
return view('simple.client.index',compact('clients'));
}
public function create(){
$actionForm = route('client.create');
return view('simple.client.form', compact('actionForm'));
}
public function store(Request $request){
$this->validate($request,[
'nom' => 'required',
'description' => 'required',
'adresse' => 'required'
]);
$client = new Client;
$client->nom = $request->nom;
$client->description = $request->description;
$client->adresse = $request->adresse;
$client->save();
return redirect(route('client.list'))->with('successMsg',"Le client est ajouté avec succès");
}
public function edit(Client $client){
$actionForm = route('client.update', ['client' => $client]);
return view('simple.client.form',compact('client','actionForm'));
}
public function update(Request $request, Client $client){
$this->validate($request,[
'nom' => 'required',
'description' => 'required',
'adresse' => 'required'
]);
$client->nom = $request->nom;
$client->description = $request->description;
$client->adresse = $request->adresse;
$client->save();
return redirect(route('client.list'))->with('successMsg',"Le client est modifié avec succès");
}
public function delete(client $client){
$client->delete();
return redirect(route('client.list'));
}
}
<file_sep><?php
namespace App\Http\Controllers\Gestionnaire;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
use App\Models\Action;
use App\Models\Anomalie;
use App\Models\Audit;
use Illuminate\Support\Facades\Auth;
class ActionController extends Controller
{
public function index(){
$actions = Action::paginate(10);
return view('quality.action.index',compact('actions'));
}
public function create(){
$actionForm = route('action.create');
return view('quality.action.form', compact('actionForm'));
}
public function store(Request $request){
$this->validate($request,[
'description' => 'required',
'designation' => 'required',
'resultat' => 'required',
'type' => 'required'
]);
$action = new Action;
$action->type = $request->type;
$action->designation = $request->designation;
$action->description = $request->description;
$action->resultat = $request->resultat;
$action->user_id = Auth::user()->id;
$action->save();
if(!empty($request->id)){
$anomalie = Anomalie::findOrFail($request->id);
return view('quality.anomalie.create', compact('anomalie'));
}
else{
if( !empty($request->idAudit)){
$audit = Audit::findOrFail($request->idAudit);
return view('quality.audit.create', compact('audit'));
}
else{
return redirect(route('action.list'))->with('successMsg',"L'action est ajoutée avec succès");
}
}
}
public function edit(Action $action){
$actionForm = route('action.update', ['action' => $action]);
return view('quality.action.form',compact('action','actionForm'));
}
public function update(Request $request, Action $action){
$this->validate($request,[
'description' => 'required',
'designation' => 'required',
'resultat' => 'required',
'type' => 'required'
]);
$action->type = $request->type;
$action->designation = $request->designation;
$action->description = $request->description;
$action->resultat = $request->resultat;
$action->user_id = Auth::user()->id;
$action->save();
return redirect(route('action.list'))->with('successMsg',"Modification de l'action avec succès");
}
public function delete(Action $action){
$action->delete();
return redirect(route('action.list'));
}
}
<file_sep><?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Regle extends Model
{
public function user(){
return $this->belongsTo('App\User');
}
public function anomalies(){
return $this->belongsToMany('App\Models\Anomalie');
}
public function produit()
{
return $this->belongsTo('App\Models\Produit');
}
public function audits()
{
return $this->belongsToMany('App\Models\Audit');
}
}
<file_sep><?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreateInspectionsTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('inspections', function (Blueprint $table) {
$table->bigIncrements('id');
$table->string('titre');
$table->integer('step')->default(1);
$table->enum('etat', ['nouveau','en cours','traité'])->default('nouveau');
$table->text('resultats')->nullable();
$table->text('commentaire')->nullable();
$table->string('productimg')->nullable();
$table->text('description')->nullable();
$table->integer('quantiteD')->nullable();
$table->unsignedBigInteger('user_id')->nullable();
$table->unsignedBigInteger('test_id')->nullable();
$table->unsignedBigInteger('lot_id')->nullable();
$table->unsignedBigInteger('anomalie_id')->nullable();
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('inspections');
}
}
<file_sep><?php
namespace App\Http\Controllers\Gestionnaire;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
use App\Models\Anomalie;
use App\Models\Lot;
use App\Models\Produit;
use App\Models\Caracteristique;
use App\Models\Alert;
use App\Models\Fournisseur;
use App\Models\Client;
use App\Models\Atelier;
use App\Models\Regle;
use App\User;
use App\Models\Examen;
use App\Models\Reponse;
use Carbon\Carbon;
use DB;
use PDF;
use App\Exports\AnomaliesExport;
use Maatwebsite\Excel\Facades\Excel;
use Illuminate\Support\Facades\Auth;
class AnomalieController extends Controller
{
public function export(){
$year = Carbon::now()->format('Y');
return (new AnomaliesExport($year))->download('Journal des Anomalies '.$year.'.xlsx');
}
public function index(){
$anomalies = Anomalie::orderBy('id','desc')->paginate(10);
return view('quality.anomalie.index',compact('anomalies'));
}
public function createFromScratch(Request $request){
return view('quality.anomalie.create');
}
public function createFrom(Request $request, $id){
$anomalie = Anomalie::findOrFail($id);
$alerts = Alert::where('anomalie_id', '=' , $id )->
where('etat', '=' , 'nouveau' )->get();
if(!empty($alerts[0])){
$alerts[0]->etat ="en cours";
$alerts[0]->save();
}
return view('quality.anomalie.create', compact('anomalie'));
}
public function postCreateStep1(Request $request){
$validatedData = $request->validate([
'titre' => 'required',
'type' => 'required',
'test' => 'required',
'quantite' => ['required','integer'],
'caracteristiquep' => 'required'
]);
if(empty($request->id)){
$anomalie = new Anomalie();
$lot_id = DB::table('lots')->insertGetId(
[ 'quantite' => $request->quantite,
'produit_id' => $request->produit,
'caracteristiquep' => $request->caracteristiquep
]
);
$anomalie->lot_id = $lot_id;
}
else{
$anomalie = Anomalie::findOrFail($request->id);
$lot_id = DB::table('lots')
->where('id', $anomalie->lot_id )
->update([
'quantite' => $request->quantite,
'produit_id' => $request->produit,
'caracteristiquep' => $request->caracteristiquep
]);
}
switch ($request->type) {
case 'Retour fournisseur':
$anomalie->fournisseur_id = $request->fournisseur;
break;
case 'Retour client':
$anomalie->client_id = $request->client;
break;
case 'Retour production':
$anomalie->atelier_id = $request->atelier;
break;
}
$anomalie->test_id = $request->test;
$anomalie->type = $request->type;
$anomalie->titre = $request->titre;
$anomalie->description = $request->description;
if($anomalie->etat != "en cours" && $anomalie->etat != "traité"){
$anomalie->etat="nouveau";
} else{
$anomalie->etat="en cours";
}
$anomalie->step = 2;
$anomalie->save();
/* Associate an alert if anomaly created from scratch */
$alerts = Alert::where('anomalie_id', '=' , $anomalie->id )->
whereNotIn('type',['Rappel'])->get();
if(empty($alerts[0])){
$alert = new Alert;
$alert->type = $anomalie->type;
$alert->description = $anomalie->description;
switch ($anomalie->type) {
case 'Retour fournisseur':
$alert->fournisseur_id = $anomalie->fournisseur_id;
break;
case 'Retour client':
$alert->client_id = $anomalie->client_id;
break;
case 'Retour production':
$alert->atelier_id = $anomalie->atelier_id;
break;
}
$alert->sent = 1;
$alert->anomalie_id = $anomalie->id;
$alert->etat = "en cours";
$alert->lot_id = $lot_id;
$alert->save();
}
$examens = Examen::where('test_id', '=' , $anomalie->test_id )->get();
return view('quality.anomalie.create', compact('anomalie','examens'));
}
public function postCreateStep2(Request $request){
$anomalie = Anomalie::findOrFail($request->id);
$result = "Les réponses des examens sont toutes correctes, Aucune erreur detecté";
if (!empty($request->ReponsesEtat)){
for ($i=0; $i < count($request->ReponsesEtat) ; $i++) {
if ($request->ReponsesEtat[$i] =="Incorrect"){
$result = "Les réponses des examens ne sont pas toutes correctes, Existence d'une anomalie !";
}
DB::table('reponses')
->updateOrInsert(
['anomalie_id' => $anomalie->id, 'examen_id' => $request->ExamensIdd[$i]],
['valeur' => $request->ReponsesValeur[$i] ,
'examen_id' => $request->ExamensIdd[$i] ,
'anomalie_id' => $anomalie->id,
'etat' => $request->ReponsesEtat[$i] ]
);
}
}
if($result == "Les réponses des examens ne sont pas toutes correctes, Existence d'une anomalie !"){
/* make something red */
}
$anomalie->etat="en cours";
$anomalie->step = 3;
$anomalie->resultats = $result;
$anomalie->save();
$examens = Examen::where('test_id', '=' , $anomalie->test_id )->get();
return view('quality.anomalie.create',compact('anomalie','examens'));
}
public function postCreateStep3(Request $request){
$anomalie = Anomalie::findOrFail($request->id);
$validatedData = $request->validate([
'productimg' => 'image|mimes:jpeg,png,jpg,gif,svg',
'diagnostique' => 'required',
'actions' => 'required'
]);
if(!empty($request->actions)){
$anomalie->actions()->attach($request->actions);
}
if($request->reparer == "on" || !empty($anomalie->reparateur_id)){
$anomalie->reparateur_id = $request->reparateur;
if(!empty($request->productimg)){
$fileName = "FR-" . time() . '.' . request()->productimg->getClientOriginalExtension();
$request->productimg->storeAs('ficheReparation', $fileName);
$anomalie->productimg = $fileName;
}
} else{
$anomalie->reparateur_id = null;
$anomalie->productImg = null;
}
$examens = Examen::where('test_id', '=' , $anomalie->test_id )->get();
$anomalie->diagnostique = $request->diagnostique;
$anomalie->step = 4;
$anomalie->save();
return view('quality.anomalie.create', compact('anomalie','examens'));
}
public function store(Request $request){
$anomalie = Anomalie::findOrFail($request->id);
$request->validate([
'cause' => 'required',
'criticite' => 'required',
'regles' => 'required'
]);
if(!empty($request->regles)){
$anomalie->regles()->attach($request->regles);
}
$anomalie->criticite = $request->criticite;
$anomalie->cause = $request->cause;
$anomalie->etat = "traité";
$anomalie->step = 1;
$anomalie->save();
/* Update state of alerts */
$alerts = Alert::where('anomalie_id', '=' , $anomalie->id )->
whereNotIn('type',['Rappel'])->
where('etat', '=' , 'en cours' )->get();
if(!empty($alerts[0])){
$alerts[0]->etat ="traité";
$alerts[0]->save();
}
return redirect(route('anomalie.dashbord'));
}
public function delete(Anomalie $anomalie){
$anomalie->delete();
return redirect(route('anomalie.dashbord'));
}
public function previous(Anomalie $anomalie){
$anomalie->step-=1;
if($anomalie->step < 1) $anomalie->step=1;
$anomalie->save();
$examens = Examen::where('test_id', '=' , $anomalie->test_id )->get();
return view('quality.anomalie.create', compact('anomalie','examens'));
}
function generate_pdf(Anomalie $anomalie) {
$pdf = PDF::loadView('pdf.ficheAnomalie', compact('anomalie'));
return $pdf->stream('Fiche.pdf');
}
public function view(Anomalie $anomalie){
return view('quality.anomalie.view',compact('anomalie'));
}
}<file_sep><?php
namespace App\Http\Controllers\Gestionnaire;
use DB;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
use App\Models\Lot;
use App\Models\Test;
use App\Models\Audit;
use App\Models\Alert;
use App\Models\Event;
use App\Models\Produit;
use App\Models\Examen;
use App\Models\Reponse;
use App\Models\Questionnaire;
use Auth;
use Redirect,Response;
use PDF;
use Carbon\Carbon;
use App\Exports\AuditsExport;
use Maatwebsite\Excel\Facades\Excel;
class AuditController extends Controller
{
public function export(){
$year = Carbon::now()->format('Y');
return (new AuditsExport($year))->download('Journal des audits '.$year.'.xlsx');
}
public function index(){
$audits = Audit::orderBy('id','desc')->paginate(10);
return view('quality.audit.index',compact('audits'));
}
public function createFrom(Request $request, $id){
$event = Event::findOrFail($id);
$event->rappel = "false"; // update rappel variable
$event->save();
$audit = Audit::where('id', '=' , $event->audit_id)->first();
if($event->alert != null){
$event->alert->etat ="en cours";
$event->alert->save();
}
return view('quality.audit.create', compact('audit'));
}
public function create(Request $request){
return view('quality.audit.create');
}
public function postCreateStep1(Request $request){
if(empty($request->id)){
$audit = new audit();
}
else{
$audit = audit::findOrFail($request->id);
$update_lot = DB::table('events')
->where('audit_id', $audit->id )
->update(['title' => $audit->titre, 'start' => $request->date ,
'end' => $request->date]);
}
$request->validate([
'titre' => 'required',
'date' => 'required',
'description' => 'required',
'procede' => 'required'
]);
$audit->titre = $request->titre;
$audit->description = $request->description;
$audit->procede_id = $request->procede;
$audit->user_id = Auth::user()->id; // Id de l'utilisateur authentifie
$audit->etat = "nouveau";
$audit->step = 2;
$audit->save();
if(empty($request->id)){
$event = new Event();
$event->title = $audit->titre;
$event->start = $request->date;
$event->end = $request->date;
$event->type = 'Audit';
$event->audit_id = $audit->id;
$event->save();
}
return view('quality.audit.create',compact('audit'));
}
public function postCreateStep2(Request $request){
$audit = Audit::findOrFail($request->id);
Questionnaire::where('audit_id', $audit->id )->delete();
if (!empty($request->IdQ) ){
for ($i=0; $i < count($request->IdQ) ; $i++) {
if ( !empty($request->IdQ[$i]) ){
DB::table('questionnaires')
->updateOrInsert(
['audit_id' => $audit->id , 'id' => $request->IdQ[$i] ],
['question' => $request->questions[$i],
'reponse' => $request->reponses[$i],
'remarque' => $request->remarques[$i],
'audit_id' => $audit->id ]
);
}
else{
$questionnaire = new Questionnaire();
$questionnaire->question = $request->questions[$i];
$questionnaire->reponse = $request->reponses[$i];
$questionnaire->remarque = $request->remarques[$i];
$questionnaire->audit_id = $audit->id;
$questionnaire->save();
}
}
}
$audit->etat = "en cours";
$audit->step = 3;
$audit->save();
return view('quality.audit.create',compact('audit'));
}
public function store(Request $request)
{
$audit = audit::findOrFail($request->id);
$request->validate([
'resultats' => 'required'
]);
if(!empty($request->actions)){
$audit->actions()->attach($request->actions);
}
if(!empty($request->regles)){
$audit->regles()->attach($request->regles);
}
$audit->resultats = $request->resultats;
if (!empty($request->commentaire)){
$audit->commentaire = $request->commentaire;
}
$audit->etat = "traité";
$audit->step = 1;
$audit->save();
return redirect(route('audit.dashbord'))->with('successMsg',"Création d'un nouveau audit");
}
public function delete(Audit $audit){
$audit->delete();
return redirect(route('audit.dashbord'));
}
public function deleteQ(Request $request, Questionnaire $questionnaire){
$audit = Audit::findOrFail($request->id);
$audit->step = 3;
$audit->save();
$questionnaire->delete();
return redirect(route('audit.create-step2'));
// return view('quality.audit.create',compact('audit'));
}
public function previous(Audit $audit){
$audit->step -= 1;
if($audit->step < 1) $audit->step = 1;
$audit->save();
return view('quality.audit.create', compact('audit'));
}
public function edit(Audit $audit){
$audit->step = 1;
$audit->etat = "en cours";
return view('quality.audit.create',compact('audit'));
}
function generate_pdf(Audit $audit) {
$pdf = PDF::loadView('pdf.ficheAudit', compact('audit'));
return $pdf->stream('Fiche.pdf');
}
public function view(Audit $audit){
return view('quality.audit.view',compact('audit'));
}
}
<file_sep><?php
use Illuminate\Database\Seeder;
use Carbon\Carbon;
class TestTableSeeder extends Seeder
{
public function run()
{
DB::table('tests')->insert([
'id' => '1',
'nom' => 'Test de fiabilité FD 3.0 (16 Go)',
'type' => 'physique',
'description' => 'Vérifier la fiabilité du flash disque de type 3.0 et capacité 16 Go',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('tests')->insert([
'id' => '2',
'nom' => 'Test électronique du câble alimentation',
'type' => 'électronique',
'description' => 'Vérifier les caractéristiques électroniques du câble alimentation',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('tests')->insert([
'id' => '3',
'nom' => 'Test des caracteristiques du câble alimentation',
'type' => 'physique',
'description' => 'Vérifier les caractéristiques du câble alimentation',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('tests')->insert([
'id' => '4',
'nom' => 'Test fonctionel pour souris',
'type' => 'fonctionel',
'description' => 'Vérifier les caractéristiques de la souris',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('tests')->insert([
'id' => '5',
'nom' => 'Test électronique du chargeur',
'type' => 'électronique',
'description' => 'Vérifier les caractéristiques électroniques du chargeur',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('tests')->insert([
'id' => '6',
'nom' => 'Test fonctionel du câble USB',
'type' => 'fonctionel',
'description' => 'Vérifier la fonctionnalité du câble USB ',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('tests')->insert([
'id' => '7',
'nom' => 'Test de fiabilité FD 3.0 (32 Go)',
'type' => 'physique',
'description' => 'Vérifier la fiabilité du flash disque de type 3.0 et capacité 32 Go',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
}
}
<file_sep><?php
namespace App\Http\Controllers\Admin;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
use Spatie\Permission\Models\Role;
use Spatie\Permission\Models\Permission;
use App\User;
class RoleController extends Controller
{
public function index(){
$roles = Role::paginate(5);
return view('admin.role.index',compact('roles'));
}
public function create(){
$permissions = Permission::all();
$action = route('role.create');
return view('admin.role.form',compact('permissions','action'));
}
public function store(Request $request){
$this->validate($request,[
'name' => ['required', 'string', 'max:255','unique:roles'],
'description' => ['required', 'string', 'max:255'],
'permissions' => 'required',
]);
$role = Role::create(['name'=>$request->name, 'description'=>$request->description]);
$role->syncPermissions($request->permissions);
$role->save();
return redirect(route('role.dashbord'))
->with('successMsg','Role créé avec succès');
}
public function edit(Role $role){
$action = route('role.update', ['role' => $role]);
$permissions = Permission::all();
return view('admin.role.form',compact('role','permissions','action'));
}
public function update(Request $request,Role $role){
$role->name = $request->name;
$role->description = $request->description;
$role->syncPermissions($request->permissions);
$role->save();
return redirect(route('role.dashbord'))
->with('successMsg','Role modifié avec succès');
}
public function delete(Role $role){
$role->delete();
return redirect(route('role.dashbord'));
}
}
<file_sep><?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreateAnomaliesTable extends Migration
{
public function up()
{
Schema::create('anomalies', function (Blueprint $table) {
$table->bigIncrements('id');
$table->text('titre')->nullable();
$table->text('description')->nullable();
$table->string('type')->nullable();
$table->double('amount', 8, 2)->nullable();
$table->enum('etat', ['nouveau','en cours','traité'])->default('nouveau');
$table->integer('step')->default(1);
$table->string('criticite')->nullable();
$table->text('cause')->nullable();
$table->text('diagnostique')->nullable();
$table->text('resultats')->nullable();
$table->string('action')->nullable(); // to be changed with table (action_anomalie)
/* one to many */
$table->string('productimg')->nullable(); // used temporary after will use (fichier table)
$table->unsignedBigInteger('lot_id'); // required
$table->unsignedBigInteger('reparateur_id')->nullable();
$table->unsignedBigInteger('test_id')->nullable();
$table->unsignedBigInteger('atelier_id')->nullable();
$table->unsignedBigInteger('fournisseur_id')->nullable();
$table->unsignedBigInteger('client_id')->nullable();
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('anomalies');
}
}
<file_sep><?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Lot extends Model
{
public function produit()
{
return $this->belongsTo('App\Models\Produit');
}
public function anomalies()
{
return $this->hasMany('App\Models\Anomalie');
}
public function alerts()
{
return $this->hasMany('App\Models\Alert');
}
public function inspections()
{
return $this->hasMany('App\Models\Inspection');
}
}
<file_sep>
$(document).ready(function () {
var SITEURL = "{{url('/')}}";
$.ajaxSetup({
headers: {
'X-CSRF-TOKEN': $('meta[name="csrf-token"]').attr('content')
}
});
var calendar = $('#calendar').fullCalendar({
header: {
left: 'prev,next,today',
center: 'title',
right: 'month,agendaWeek,agendaDay,list'
},
editable: true,
events: SITEURL + "/fullcalendareventmaster",
displayEventTime: true,
editable: true,
eventRender: function (event, element, view) {
if (event.allDay === 'true') {
event.allDay = false;
} else {
event.allDay = false;
}
},
selectable: true,
selectHelper: true,
select: function (start, end, allDay) {
$('#modalAddEvent').modal();
$("#AddEventForm").submit(function(){
var start = $.fullCalendar.formatDate(start, "Y-MM-DD HH:mm:ss");
var end = $.fullCalendar.formatDate(end, "Y-MM-DD HH:mm:ss");
var title =$('#title').val();
//var heure =$('#heure').val();
var type = $('#type').find('option:selected').text();
$.ajax({
url: SITEURL + "/fullcalendareventmaster/create",
data: 'title=' + title + '&type=' + type + '&start=' + start + '&end=' + end,
type: "POST",
success: function (data) {
$('#modalAddEvent').modal('hide');
swal({
title: "Bravo!",
text: "Planification d'un évenement",
type: "success",
showConfirmButton: true
});
},
error: function(data){
$('#modalAddEvent').modal('hide');
sweetAlert(
"Erreur...",
data,
"error");
}
});
calendar.fullCalendar('renderEvent',
{
title: title,
start: start,
end: end,
allDay: allDay
},
true
);
});
calendar.fullCalendar('unselect');
},
eventDrop: function (event, delta) {
var start = $.fullCalendar.formatDate(event.start, "Y-MM-DD HH:mm:ss");
var end = $.fullCalendar.formatDate(event.end, "Y-MM-DD HH:mm:ss");
$.ajax({
url: SITEURL + '/fullcalendareventmaster/update',
data: 'title=' + event.title + '&type=' + type + '&start=' + start + '&end=' + end + '&id=' + event.id,
type: "POST",
success: function (response) {
/* swal({
title: "Bravo!",
text: "Date d'évenement modifiée",
type: "success",
showConfirmButton: true
}); */
}
});
},
eventClick: function (event) {
swal({
title: "Voulez-vous supprimer cet évenement ?",
text: "",
type: "warning",
showCancelButton: true,
confirmButtonColor: "#DD6B55",
confirmButtonText: "Oui, supprimer",
cancelButtonText: "Non, annuler",
closeOnConfirm: false,
closeOnCancel: false
},
function(isConfirm){
if (isConfirm) {
$.ajax({
type: "POST",
url: SITEURL + '/fullcalendareventmaster/delete',
data: "&id=" + event.id,
success: function (response) {
if(parseInt(response) > 0) {
$('#calendar').fullCalendar('removeEvents', event.id);
swal("Suppression !!", "Votre évenement a été supprimer !!", "success");
}
}
});
}
else {
swal("Annulation !!", "Votre évenement existe encore", "error");
}
});
}
});
});
<file_sep><?php
use Illuminate\Database\Seeder;
use Carbon\Carbon;
class ClientTableSeeder extends Seeder
{
/**
* Run the database seeds.
*
* @return void
*/
public function run()
{
DB::table('clients')->insert([
'id' => '1',
'nom' => '<NAME>',
'description' => '',
'adresse' => 'BP 09 Oued Smar – Alger',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('clients')->insert([
'id' => '2',
'nom' => '<NAME>',
'description' => '',
'adresse' => '45,che<NAME>, 16040 hussein Dey, Alger',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('clients')->insert([
'id' => '3',
'nom' => 'BZA Hardware',
'description' => '',
'adresse' => 'Cité abdouni boualem lot n° 1, <NAME>ida,Alger',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('clients')->insert([
'id' => '4',
'nom' => '<NAME>',
'description' => '',
'adresse' => 'Siège social RN n°5, Cinq Maisons, Mohammadia 16130 Alger',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('clients')->insert([
'id' => '5',
'nom' => '<NAME>',
'description' => '',
'adresse' => 'LOT N° 1 groupe 13 El hamiz, Dar El Beida Alger',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('clients')->insert([
'id' => '6',
'nom' => '<NAME>',
'description' => '',
'adresse' => 'Local N°01 Route de Sebal N°10 El Achour,Alger',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('clients')->insert([
'id' => '7',
'nom' => 'ISI',
'description' => '',
'adresse' => 'Lotissement kaouch Villa N° 8 (deriere AGB Bank) <NAME>, Alger',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('clients')->insert([
'id' => '8',
'nom' => '<NAME>',
'description' => '',
'adresse' => 'Zone d’activités Mesra, Mostaganem',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('clients')->insert([
'id' => '9',
'nom' => 'COMPUSTROE',
'description' => '',
'adresse' => 'Lotissement boursas villa N°21 HYDRA ALGER',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
DB::table('clients')->insert([
'id' => '10',
'nom' => 'RedFabriq',
'description' => '',
'adresse' => '89, Lot Vincent II Bouzareah, Alger',
'created_at' => Carbon::now()->format('Y-m-d H:i:s'),
'updated_at' => Carbon::now()->format('Y-m-d H:i:s')
]);
}
}
<file_sep><?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Action extends Model
{
public function user()
{
return $this->belongsTo('App\User');
}
public function attributs()
{
return $this->hasMany('App\Models\Attribut');
}
public function audits()
{
return $this->belongsToMany('App\Models\Audit');
}
public function anomalies()
{
return $this->belongsToMany('App\Models\Anomalie');
}
}
<file_sep><?php
namespace App\Http\Controllers\Admin;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
use Spatie\Permission\Models\Role;
use Spatie\Permission\Models\Permission;
use App\User;
class PermissionController extends Controller
{
public function index(){
$permissions = Permission::paginate(10);
return view('admin.permission.index',compact('permissions'));
}
public function create(){
$roles = Role::all();
$action = route('permission.create');
return view('admin.permission.form',compact('roles','action'));
}
public function store(Request $request){
$this->validate($request,[
'name' => ['required', 'string', 'max:255','unique:permissions'],
'description' => ['required', 'string', 'max:255'],
]);
$permission = Permission::create([
'name'=> $request->name,
'description'=> $request->description
]);
//$permission->syncRoles($request->roles);
/*foreach($request->models as $model) {
$model->givePermissionTo($permission->name);
}*/
$permission->syncRoles($request->roles);
$query = $permission->save();
return redirect(route('permission.dashbord'))
->with('successMsg','Permission créé avec succès');
}
public function edit(Permission $permission){
$action = route('permission.update', ['permission' => $permission]);
$roles = Role::all();
return view('admin.permission.form',compact('permission','roles','action'));
}
public function update(Request $request,Permission $permission){
$permission->name = $request->name;
$permission->description = $request->description;
$permission->syncRoles($request->roles);
$permission->save();
return redirect(route('permission.dashbord'))
->with('successMsg','Permission modifiée avec succès');
}
public function delete(Permission $permission){
$permission->delete();
return redirect(route('permission.dashbord'));
}
}
<file_sep><?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreateFichiersTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('fichiers', function (Blueprint $table) {
$table->bigIncrements('id');
$table->string('nom');
$table->string('type');
$table->string('url',6000);
$table->unsignedBigInteger('user_id')->nullable(); // the one who uploaded it or the user's avatar
$table->unsignedBigInteger('anomalie_id')->nullable();
$table->unsignedBigInteger('produit_id')->nullable();
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('fichiers');
}
}
<file_sep><?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Atelier extends Model
{
protected $fillable = [
'nom' , 'metier', 'description'
];
public function audits()
{
return $this->hasMany('App\Models\Audit');
}
public function procedes()
{
return $this->hasMany('App\Models\Procede');
}
public function anomalies()
{
return $this->hasMany('App\Models\Anomalie');
}
public function alerts()
{
return $this->hasMany('App\Models\Alert');
}
}
<file_sep><?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Inspection extends Model
{
public function user()
{
return $this->belongsTo('App\user');
}
public function lot()
{
return $this->belongsTo('App\Models\Lot');
}
public function anomalie()
{
return $this->belongsTo('App\Models\Anomalie');
}
public function attributs()
{
return $this->hasMany('App\Models\Attribut');
}
public function reponses()
{
return $this->hasMany('App\Models\Reponse');
}
public function test()
{
return $this->belongsTo('App\Models\Test');
}
public function event()
{
return $this->belongsTo('App\Models\Event');
}
}
| 9273813abc8646a7b0754c4869af3309b554ec4a | [
"JavaScript",
"PHP"
] | 68 | PHP | LamissAmrouch/ProQuality | bcc3c045f89fc5fe34d895b8ab2365fccd2e78b3 | 398e2cf1dfb87e022a8ec5e430b47a0f6cd09253 | |
refs/heads/master | <file_sep>require File.expand_path(File.join(File.dirname(__FILE__), '..', 'spec_helper'))
describe "Lexing expressions" do
it "should have the correct tokens for simple addition" do
subject = MathEngine::Lexer.new("1 + 1")
expect(subject.next).to eq(Lexr::Token.number(1))
expect(subject.next).to eq(Lexr::Token.addition('+'))
expect(subject.next).to eq(Lexr::Token.number(1))
end
it "should have the correct tokens for simple addition without whitespace" do
subject = MathEngine::Lexer.new("1+1")
expect(subject.next).to eq(Lexr::Token.number(1))
expect(subject.next).to eq(Lexr::Token.addition('+'))
expect(subject.next).to eq(Lexr::Token.number(1))
end
it "should have the correct tokens for simple addition without whitespace" do
subject = MathEngine::Lexer.new("-10*(-3--2)")
expect(subject.next).to eq(Lexr::Token.number(-10))
expect(subject.next).to eq(Lexr::Token.multiplication("*"))
expect(subject.next).to eq(Lexr::Token.open_parenthesis("("))
expect(subject.next).to eq(Lexr::Token.number(-3))
expect(subject.next).to eq(Lexr::Token.subtraction("-"))
expect(subject.next).to eq(Lexr::Token.number(-2))
expect(subject.next).to eq(Lexr::Token.close_parenthesis(")"))
expect(subject.next).to eq(Lexr::Token.end)
end
it "should do this expression properly" do
subject = MathEngine::Lexer.new("(3+2)-2")
expect(subject.next).to eq(Lexr::Token.open_parenthesis("("))
expect(subject.next).to eq(Lexr::Token.number(3))
expect(subject.next).to eq(Lexr::Token.addition("+"))
expect(subject.next).to eq(Lexr::Token.number(2))
expect(subject.next).to eq(Lexr::Token.close_parenthesis(")"))
expect(subject.next).to eq(Lexr::Token.subtraction("-"))
expect(subject.next).to eq(Lexr::Token.number(2))
expect(subject.next).to eq(Lexr::Token.end)
end
end
<file_sep>require File.expand_path(File.join(File.dirname(__FILE__), '..', 'spec_helper'))
describe "Evaluating expressions" do
it "should have the correct value for some simple calculations" do
expect(evaluate("1 + 1")).to eq(2)
expect(evaluate("2 - 1")).to eq(1)
expect(evaluate("2 * 2")).to eq(4)
expect(evaluate("4 / 2")).to eq(2)
expect(evaluate("10 * 2 + 4")).to eq(24)
expect(evaluate("10 * (2 + 4)")).to eq(60)
expect(evaluate("10 + 10 + 10 + 10")).to eq(40)
expect(evaluate("10 * 10 + 5 / 5")).to eq(101)
expect(evaluate("3.14159 * (200 / 180) + 1")).to be_within(0.001).of(4.49)
expect(evaluate("10 * (3 * 2) + (55 - 5) / (2.5 * 2)")).to eq(70)
expect(evaluate("10 * (3 * 2) + (55 - 5) / (2.5 * (3 + 1))")).to eq(65)
expect(evaluate("2 * 2 ^ 5")).to eq(64)
expect(evaluate("(2 * 2) ^ 5")).to eq(1024)
expect(evaluate("2 * 2 % 5")).to eq(4)
expect(evaluate("2 * 4 / 3")).to be_within(0.001).of(2.66666666666667)
end
it "should have the correct values when using variables" do
subject = MathEngine.new
# expect(subject.evaluate("x = 1 * 2")).to eq(2)
# expect(subject.evaluate("x + 1")).to eq(3)
expect(subject.evaluate("i = 2 * 2")).to eq(4)
expect(subject.evaluate("i + 1")).to eq(5)
end
it "should be able to call functions and use the result in calculations" do
subject = MathEngine.new
subject.context.define(:double_it, lambda { |x| x * 2 })
subject.context.define(:add_em, lambda { |x, y| x + y })
expect(subject.evaluate("10 * double_it(2)")).to eq(40)
expect(subject.evaluate("10 * double_it(2 * 4 / 3)")).to be_within(0.001).of(53.3333333334)
expect(subject.evaluate("10 * double_it(2 * 4 / 3 + (5 ^ 5))")).to be_within(0.001).of(62553.333333)
expect(subject.evaluate("double_it(5) + add_em(10, add_em(2.5, 2.5)) + 5 + add_em(20, 20)")).to eq(70)
end
end
<file_sep>#require "mathn"
require "bigdecimal"
require_relative "errors"
require_relative "lexer"
require_relative "parser"
require_relative "context"
require_relative "evaluators/finders"
require_relative "evaluators/calculate"
class MathEngine
DEFAULT_OPTIONS = {evaluator: :calculate,
case_sensitive: true}
def initialize(opts = {})
@opts = DEFAULT_OPTIONS.merge(opts)
@opts[:context] = Context.new(@opts) unless @opts[:context]
end
def evaluate(expression)
evaluator = MathEngine::Evaluators.find_by_name(@opts[:evaluator]).new(context)
Parser.new(Lexer.new(expression)).parse.evaluate(evaluator)
end
def context
@opts[:context]
end
end
<file_sep>require File.expand_path(File.join(File.dirname(__FILE__), 'nodes'))
class MathEngine
class Parser
def initialize(lexer)
@lexer = lexer
end
def parse
#statement = { <identifier> <assignment> } expression <end>
#expression = term { ( <addition> | <subtraction> ) term }
#term = exp { ( <multiplication> | <division> ) exp }
#exp = factor { ( <exponent> | <modulus> ) factor }
#factor = <call> | <identifier> | <number> | ( <open_parenthesis> expression <close_parenthesis> )
#call = <identifier> <open_parenthesis> { call_parameter } <close_parenthesis>
#call_parameter = <expression> { <comma> call_parameter }
statement
end
private
def statement
next!
if current.type == :identifier && peek.type == :assignment
variable_name = current.value
next!
expect_current :assignment
next!
result = MathEngine::AssignmentNode.new(MathEngine::IdentifierNode.new(variable_name), expression)
else
result = expression
end
next!
expect_current :end
result
end
def expression
left = term
result = nil
while [:addition, :subtraction].include? current.type
node_type = current.type == :addition ? MathEngine::AdditionNode : MathEngine::SubtractionNode
next!
left = node_type.new(left, term)
end
result = MathEngine::ExpressionNode.new(result || left)
result
end
def term
left = exp
result = nil
while [:multiplication, :division].include? current.type
node_type = current.type == :multiplication ? MathEngine::MultiplicationNode : MathEngine::DivisionNode
next!
left = node_type.new(left, exp)
end
result || left
end
def exp
left = factor
result = nil
while [:exponent, :modulus].include? current.type
node_type = current.type == :exponent ? MathEngine::ExponentNode : MathEngine::ModulusNode
next!
left = node_type.new(left, factor)
end
result || left
end
def factor
if current.type == :number
result = MathEngine::LiteralNumberNode.new(current.value)
next!
return result
elsif current.type == :identifier
result = peek.type == :open_parenthesis ? call : MathEngine::IdentifierNode.new(current.value)
next!
return result
end
expect_current :open_parenthesis, "number, variable or open_parenthesis"
next!
result = MathEngine::ParenthesisedExpressionNode.new(expression)
expect_current :close_parenthesis
next!
result
end
def call
expect_current :identifier
function_name = current.value
next!
expect_current :open_parenthesis
next!
result = MathEngine::FunctionCallNode.new(function_name, current.type == :close_parenthesis ? nil : call_parameter)
expect_current :close_parenthesis
result
end
def call_parameter
left = expression
right = nil
if current.type == :comma
next!
right = call_parameter
end
MathEngine::ParametersNode.new(left, right)
end
def current
@lexer.current
end
def peek
@lexer.peek
end
def next!
@lexer.next
end
def expect_current(type, friendly = nil)
raise MathEngine::ParseError.new("Unexpected #{current}, expected: #{friendly ? friendly : type}") unless current.type == type
end
end
end
<file_sep># MathEngine
MathEngine is a lightweight mathematical expression parser and evaluator. It currently handles addition, subtraction, multiplication, division, exponent, modulus and the use of variables and pre-defined functions.
Install with
gem install math_engine
The only dependency is [lexr](http://github.com/michaelbaldry/lexr), which is really lightweight and has no external dependencies.
## An example: Expressions
require 'rubygems'
require 'math_engine'
engine = MathEngine.new
puts "#{engine.evaluate("x = 10 * (3 * 2) + (55 - 5) / (2.5 * (3 + 1))")}"
puts "#{engine.evaluate("x + 5")}"
results in an output of
65.0
70.0
extending is easy using functions, you can add single functions using MathEngine.define
engine.context.define :add_em do |x, y|
x + y
end
or you can write all your functions in a class and add the class
class SomeFunctions
def add_em(x, y)
x + y
end
end
engine.context.include_library SomeFunctions.new
and calling them with
engine.evaluate("1 + 1 + add_em(2, 2)")
All functions are pulled in from the built in Math class by default, so all the standard ruby math functions are available (cos, sin, tan etc)
if you missed a closing parenthesis, had an operator where it wasn't meant to be, you might get something like this:
Unexpected multiplication(*), expected: number, variable name or open_parenthesis
and that is pretty much every feature so far. Please let me know of any bugs or additions that you'd like to see!
## Contributors
<NAME> (mdelaossa): Handling of complex numbers and upgraded to work with 1.9.X
## License
See the LICENSE file included with the distribution for licensing and
copyright details.
<file_sep>require File.expand_path(File.join(File.dirname(__FILE__), '..', 'spec_helper'))
describe "Contextual scope for variables and functions" do
it "should be able to set a variable and get it again" do
subject = MathEngine::Context.new
subject.set("test", "abc123")
expect(subject.get("test")).to eq("abc123")
end
it "should be able to change a variable and get it again" do
subject = MathEngine::Context.new
subject.set("test", "abc123")
subject.set("test", "abc12345")
expect(subject.get("test")).to eq("abc12345")
end
it "should be able to set a constant (all uppercase) and get it again" do
subject = MathEngine::Context.new
subject.set("PI", 3.14159)
expect(subject.get("PI")).to eq(3.14159)
end
it "should not be possible to set a constant (all uppercase) more than once" do
subject = MathEngine::Context.new
subject.set("PI", 3.14159)
expect { subject.set("PI", 3.2) }.to raise_error MathEngine::UnableToModifyConstantError
end
it "should diferentiate between variables and constants" do
subject = MathEngine::Context.new
subject.set("blah", 123)
subject.set("PI", 3.14159)
expect(subject.variables).to eq(["blah"])
expect(subject.constants).to eq(["PI"])
end
it "should raise an error if a function is called with the wrong number of arguments" do
subject = MathEngine::Context.new
subject.define :abc, lambda { |x, y, z| x + y + z }
expect { subject.call(:abc, 123) }.to raise_error ArgumentError
end
it "should be possible to define a function with a lambda and call it" do
subject = MathEngine::Context.new
subject.define(:double_it, lambda { |x| x * 2 })
expect(subject.call(:double_it, 2)).to eq(4)
subject.define(:add_em, lambda { |x, y| x + y })
expect(subject.call(:add_em, 2, 2)).to eq(4)
end
it "should be possible to define a function with a block and call it" do
subject = MathEngine::Context.new
subject.define :double_it do |x|
x * 2
end
expect(subject.call(:double_it, 2)).to eq(4)
subject.define :add_em do |x, y|
x + y
end
expect(subject.call(:add_em, 2, 2)).to eq(4)
end
it "should be able to pull in a library and use its functions" do
subject = MathEngine::Context.new
Blah = Class.new do
def test
12345
end
end
subject.include_library Blah.new
expect(subject.call(:test)).to eq(12345)
end
it "should be able to pull in a library and use its functions, even if they are dynamic, via method_missing" do
subject = MathEngine::Context.new
Blah = Class.new do
def method_missing(sym, *args, &block)
return 12345 if sym == :test_12345
raise NoMethodError(sym)
end
def respond_to_missing?(sym, include_private)
sym == :test_12345
end
end
subject.include_library Blah.new
expect(subject.call(:test_12345)).to eq(12345)
end
it "should be able to use functions from Math by default" do
subject = MathEngine::Context.new
expect(subject.call(:sin, 0.5)).to be_within(0.001).of(0.4794255386)
end
end
describe "Contextual scope for variables and functions with case sensetivity turned off" do
it "should be able to set a variable and get it again" do
subject = MathEngine::Context.new(case_sensitive: false)
subject.set("teSt", "abc123")
expect(subject.get("test")).to eq("abc123")
end
it "should be able to change a variable and get it again" do
subject = MathEngine::Context.new(case_sensitive: false)
subject.set("tesT", "abc123")
subject.set("test", "abc12345")
expect(subject.get("test")).to eq("abc12345")
end
it "should be able to set a constant (all uppercase) and get it again" do
subject = MathEngine::Context.new(case_sensitive: false)
subject.set("PI", 3.14159)
expect(subject.get("Pi")).to eq(3.14159)
end
it "should not be possible to set a constant (all uppercase) more than once" do
subject = MathEngine::Context.new(case_sensitive: false)
subject.set("PI", 3.14159)
expect { subject.set("Pi", 3.2) }.to raise_error MathEngine::UnableToModifyConstantError
end
it "should diferentiate between variables and constants" do
subject = MathEngine::Context.new(case_sensitive: false)
subject.set("blah", 123)
subject.set("PI", 3.14159)
expect(subject.variables).to eq(["blah"])
expect(subject.constants).to eq(["PI"])
end
end
<file_sep># -*- encoding: utf-8 -*-
# stub: math_engine 0.7.0 ruby lib
Gem::Specification.new do |s|
s.name = "math_engine".freeze
s.version = "0.7.0"
s.required_rubygems_version = Gem::Requirement.new(">= 0".freeze) if s.respond_to? :required_rubygems_version=
s.require_paths = ["lib".freeze]
s.authors = ["<NAME>".freeze]
s.date = "2017-02-24"
s.description = "Lightweight matematical expression parser that is easy to extend".freeze
s.email = "<EMAIL>".freeze
s.extra_rdoc_files = ["README.md".freeze]
s.files = ["README.md".freeze, "lib/context.rb".freeze, "lib/errors.rb".freeze, "lib/evaluators/calculate.rb".freeze, "lib/evaluators/finders.rb".freeze, "lib/lexer.rb".freeze, "lib/math_engine.rb".freeze, "lib/nodes.rb".freeze, "lib/parser.rb".freeze]
s.homepage = "http://tech.buyapowa.com".freeze
s.rdoc_options = ["--main".freeze, "README.md".freeze]
s.rubygems_version = "2.5.2".freeze
s.summary = "Lightweight mathematical expression parser".freeze
if s.respond_to? :specification_version then
s.specification_version = 4
if Gem::Version.new(Gem::VERSION) >= Gem::Version.new('1.2.0') then
s.add_runtime_dependency(%q<lexr>.freeze, [">= 0.4.0"])
s.add_development_dependency(%q<rspec>.freeze, [">= 0"])
else
s.add_dependency(%q<lexr>.freeze, [">= 0.4.0"])
s.add_dependency(%q<rspec>.freeze, [">= 0"])
end
else
s.add_dependency(%q<lexr>.freeze, [">= 0.4.0"])
s.add_dependency(%q<rspec>.freeze, [">= 0"])
end
end
<file_sep>class MathEngine
module Evaluators
class Calculate
def initialize(context)
@context = context
end
def literal_number(node)
node.value
end
def expression(node)
node.left.evaluate(self)
end
def parenthesised_expression(node)
expression(node)
end
def identifier(node)
@context.get node.value
end
def assignment(node)
result = node.right.evaluate(self)
@context.set(node.left.value, result)
result
end
def addition(node)
node.left.evaluate(self) + node.right.evaluate(self)
end
def subtraction(node)
node.left.evaluate(self) - node.right.evaluate(self)
end
def multiplication(node)
node.left.evaluate(self) * node.right.evaluate(self)
end
def division(node)
node.left.evaluate(self) / node.right.evaluate(self)
end
def exponent(node)
node.left.evaluate(self) ** node.right.evaluate(self)
end
def modulus(node)
node.left.evaluate(self) % node.right.evaluate(self)
end
def function_call(node)
parameters = node.right ? node.right.to_a.collect { |p| p.evaluate(self) } : []
@context.call(node.left, *parameters)
end
def parameters(node)
node.left.evaluate(self)
end
end
end
end<file_sep>require "lexr"
class MathEngine
Lexer = Lexr.that {
ignores /\s/ => :whitespace
legal_place_for_binary_operator = lambda { |prev| [:addition,
:subtraction,
:multiplication,
:division,
:open_parenthesis,
:start].include? prev.type }
matches ',' => :comma
matches '=' => :assignment
matches '+' => :addition, unless: legal_place_for_binary_operator
matches '-' => :subtraction, unless: legal_place_for_binary_operator
matches '*' => :multiplication, unless: legal_place_for_binary_operator
matches '/' => :division, unless: legal_place_for_binary_operator
matches '^' => :exponent, unless: legal_place_for_binary_operator
matches '%' => :modulus, unless: legal_place_for_binary_operator
matches '(' => :open_parenthesis
matches ')' => :close_parenthesis
matches /[a-z][a-z0-9_]*/i => :identifier, convert_with: lambda { |v| v.to_sym }
matches /([-+]?(\d+\.?\d*|\d*\.?\d+)([Ee][-+]?[0-2]?\d{1,2})?[r]?|[-+]?((\d+\.?\d*|\d*\.?\d+)([Ee][-+]?[0-2]?\d{1,2})?)?[i]|[-+]?(\d+\.?\d*|\d*\.?\d+)([Ee][-+]?[0-2]?\d{1,2})?[r]?[-+]((\d+\.?\d*|\d*\.?\d+)([Ee][-+]?[0-2]?\d{1,2})?)?[i])/ => :number, convert_with: lambda { |v| BigDecimal(v) }
}
end
<file_sep>source "https://rubygems.org"
gem "lexr", ">= 0.4.0"
group :development, :test do
gem "rspec", "~> 3.5.0"
gem "rake"
end
<file_sep>require File.expand_path(File.join(File.dirname(__FILE__), '..', 'spec_helper'))
describe "Parsing expressions" do
it "should have the correct ast for simple addition" do
subject = build_ast("1 + 2")
expect(subject.class).to eq(MathEngine::ExpressionNode)
expect(subject.left.class).to eq(MathEngine::AdditionNode)
expect(subject.left.left.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.left.value).to eq(1)
expect(subject.left.right.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.right.value).to eq(2)
end
it "should have the correct ast for stringed addition" do
subject = build_ast("1 + 2 + 3")
expect(subject.class).to eq(MathEngine::ExpressionNode)
expect(subject.left.class).to eq(MathEngine::AdditionNode)
expect(subject.left.left.class).to eq(MathEngine::AdditionNode)
expect(subject.left.left.left.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.left.left.value).to eq(1)
expect(subject.left.left.right.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.left.right.value).to eq(2)
expect(subject.left.right.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.right.value).to eq(3)
end
it "should handle precedence of multiplication correctly" do
subject = build_ast("10 * 2 - 3")
expect(subject.class).to eq(MathEngine::ExpressionNode)
expect(subject.left.class).to eq(MathEngine::SubtractionNode)
expect(subject.left.left.class).to eq(MathEngine::MultiplicationNode)
expect(subject.left.left.left.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.left.left.value).to eq(10)
expect(subject.left.left.right.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.left.right.value).to eq(2)
expect(subject.left.right.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.right.value).to eq(3)
end
it "should handle precedence of division correctly" do
subject = build_ast("10 / 2 - 3")
expect(subject.class).to eq(MathEngine::ExpressionNode)
expect(subject.left.class).to eq(MathEngine::SubtractionNode)
expect(subject.left.left.class).to eq(MathEngine::DivisionNode)
expect(subject.left.left.left.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.left.left.value).to eq(10)
expect(subject.left.left.right.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.left.right.value).to eq(2)
expect(subject.left.right.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.right.value).to eq(3)
end
it "should handle precedence of enclosed expressions" do
subject = build_ast("10 * (2 + 3)")
expect(subject.class).to eq(MathEngine::ExpressionNode)
expect(subject.left.class).to eq(MathEngine::MultiplicationNode)
expect(subject.left.left.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.left.value).to eq(10)
expect(subject.left.right.class).to eq(MathEngine::ParenthesisedExpressionNode)
expect(subject.left.right.left.class).to eq(MathEngine::ExpressionNode)
expect(subject.left.right.left.left.class).to eq(MathEngine::AdditionNode)
expect(subject.left.right.left.left.left.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.right.left.left.left.value).to eq(2)
expect(subject.left.right.left.left.right.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.right.left.left.right.value).to eq(3)
end
it "should handle assignment of expressions" do
subject = build_ast("x = 10 * 10")
expect(subject.class).to eq(MathEngine::AssignmentNode)
expect(subject.left.class).to eq(MathEngine::IdentifierNode)
expect(subject.left.value).to eq(:x)
expect(subject.right.class).to eq(MathEngine::ExpressionNode)
expect(subject.right.left.class).to eq(MathEngine::MultiplicationNode)
expect(subject.right.left.left.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.right.left.left.value).to eq(10)
expect(subject.right.left.right.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.right.left.right.value).to eq(10)
end
it "should handle exponents" do
subject = build_ast("1 ^ 5")
expect(subject.class).to eq(MathEngine::ExpressionNode)
expect(subject.left.class).to eq(MathEngine::ExponentNode)
expect(subject.left.left.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.left.value).to eq(1)
expect(subject.left.right.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.right.value).to eq(5)
end
it "should handle exponent precendence as higher than multiplication" do
subject = build_ast("2 * 2 ^ 5")
expect(subject.class).to eq(MathEngine::ExpressionNode)
expect(subject.left.class).to eq(MathEngine::MultiplicationNode)
expect(subject.left.left.class).to eq(MathEngine::LiteralNumberNode)
subject.left.left.value == 2
expect(subject.left.right.class).to eq(MathEngine::ExponentNode)
expect(subject.left.right.left.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.right.left.value).to eq(2)
expect(subject.left.right.right.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.right.right.value).to eq(5)
end
it "should handle modulus precendence as higher than multiplication" do
subject = build_ast("2 * 2 % 5")
expect(subject.class).to eq(MathEngine::ExpressionNode)
expect(subject.left.class).to eq(MathEngine::MultiplicationNode)
expect(subject.left.left.class).to eq(MathEngine::LiteralNumberNode)
subject.left.left.value == 2
expect(subject.left.right.class).to eq(MathEngine::ModulusNode)
expect(subject.left.right.left.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.right.left.value).to eq(2)
expect(subject.left.right.right.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.right.right.value).to eq(5)
end
it "should handle function calls with no parameters" do
subject = build_ast("2 * sin()")
expect(subject.class).to eq(MathEngine::ExpressionNode)
expect(subject.left.class).to eq(MathEngine::MultiplicationNode)
expect(subject.left.left.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.left.value).to eq(2)
expect(subject.left.right.class).to eq(MathEngine::FunctionCallNode)
expect(subject.left.right.left).to eq(:sin)
end
it "should handle function calls with 1 literal parameter" do
subject = build_ast("2 * sin(2)")
expect(subject.class).to eq(MathEngine::ExpressionNode)
expect(subject.left.class).to eq(MathEngine::MultiplicationNode)
expect(subject.left.left.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.left.value).to eq(2)
expect(subject.left.right.class).to eq(MathEngine::FunctionCallNode)
expect(subject.left.right.left).to eq(:sin)
expect(subject.left.right.right.class).to eq(MathEngine::ParametersNode)
expect(subject.left.right.right.left.class).to eq(MathEngine::ExpressionNode)
expect(subject.left.right.right.left.left.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.right.right.left.left.value).to eq(2)
end
it "should handle function calls with 2 literal parameter" do
subject = build_ast("2 * something(1, 2)")
expect(subject.class).to eq(MathEngine::ExpressionNode)
expect(subject.left.class).to eq(MathEngine::MultiplicationNode)
expect(subject.left.left.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.left.value).to eq(2)
expect(subject.left.right.class).to eq(MathEngine::FunctionCallNode)
expect(subject.left.right.left).to eq(:something)
expect(subject.left.right.right.class).to eq(MathEngine::ParametersNode)
expect(subject.left.right.right.left.class).to eq(MathEngine::ExpressionNode)
expect(subject.left.right.right.left.left.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.right.right.left.left.value).to eq(1)
expect(subject.left.right.right.right.class).to eq(MathEngine::ParametersNode)
expect(subject.left.right.right.right.left.class).to eq(MathEngine::ExpressionNode)
expect(subject.left.right.right.right.left.left.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.right.right.right.left.left.value).to eq(2)
end
it "should handle function calls with more literal parameter" do
subject = build_ast("2 * something(1, 2, 3)")
expect(subject.class).to eq(MathEngine::ExpressionNode)
expect(subject.left.class).to eq(MathEngine::MultiplicationNode)
expect(subject.left.left.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.left.value).to eq(2)
expect(subject.left.right.class).to eq(MathEngine::FunctionCallNode)
expect(subject.left.right.left).to eq(:something)
expect(subject.left.right.right.class).to eq(MathEngine::ParametersNode)
expect(subject.left.right.right.left.class).to eq(MathEngine::ExpressionNode)
expect(subject.left.right.right.left.left.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.right.right.left.left.value).to eq(1)
expect(subject.left.right.right.right.class).to eq(MathEngine::ParametersNode)
expect(subject.left.right.right.right.left.class).to eq(MathEngine::ExpressionNode)
expect(subject.left.right.right.right.left.left.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.right.right.right.left.left.value).to eq(2)
expect(subject.left.right.right.right.right.class).to eq(MathEngine::ParametersNode)
expect(subject.left.right.right.right.right.left.class).to eq(MathEngine::ExpressionNode)
expect(subject.left.right.right.right.right.left.left.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.right.right.right.right.left.left.value).to eq(3)
end
it "should handle function calls with 1 expression parameter" do
subject = build_ast("sin(2 * 4)")
expect(subject.class).to eq(MathEngine::ExpressionNode)
expect(subject.left.class).to eq(MathEngine::FunctionCallNode)
expect(subject.left.left).to eq(:sin)
expect(subject.left.right.class).to eq(MathEngine::ParametersNode)
expect(subject.left.right.left.class).to eq(MathEngine::ExpressionNode)
expect(subject.left.right.left.left.class).to eq(MathEngine::MultiplicationNode)
expect(subject.left.right.left.left.left.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.right.left.left.left.value).to eq(2)
expect(subject.left.right.left.left.right.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.right.left.left.right.value).to eq(4)
end
it "should handle nested function calls" do
subject = build_ast("sin(2 * sin(4))")
expect(subject.class).to eq(MathEngine::ExpressionNode)
expect(subject.left.class).to eq(MathEngine::FunctionCallNode)
expect(subject.left.left).to eq(:sin)
expect(subject.left.right.class).to eq(MathEngine::ParametersNode)
expect(subject.left.right.left.class).to eq(MathEngine::ExpressionNode)
expect(subject.left.right.left.left.class).to eq(MathEngine::MultiplicationNode)
expect(subject.left.right.left.left.left.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.right.left.left.left.value).to eq(2)
expect(subject.left.right.left.left.right.class).to eq(MathEngine::FunctionCallNode)
expect(subject.left.right.left.left.right.left).to eq(:sin)
expect(subject.left.right.left.left.right.right.class).to eq(MathEngine::ParametersNode)
expect(subject.left.right.left.left.right.right.left.class).to eq(MathEngine::ExpressionNode)
expect(subject.left.right.left.left.right.right.left.left.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.right.left.left.right.right.left.left.value).to eq(4)
end
it "should parse numbers correctly when no whitespace separates them (bug)" do
subject = build_ast("10 * (2+3)")
expect(subject.class).to eq(MathEngine::ExpressionNode)
expect(subject.left.class).to eq(MathEngine::MultiplicationNode)
expect(subject.left.left.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.left.value).to eq(10)
expect(subject.left.right.class).to eq(MathEngine::ParenthesisedExpressionNode)
expect(subject.left.right.left.class).to eq(MathEngine::ExpressionNode)
expect(subject.left.right.left.left.class).to eq(MathEngine::AdditionNode)
expect(subject.left.right.left.left.left.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.right.left.left.left.value).to eq(2)
expect(subject.left.right.left.left.right.class).to eq(MathEngine::LiteralNumberNode)
expect(subject.left.right.left.left.right.value).to eq(3)
end
end
describe "Invalid syntax" do
it "should raise an exception when an expression is incomplete" do
expect { build_ast("1 *") }.to raise_error MathEngine::ParseError
end
it "should raise an exception when an extra operator is specified" do
expect { build_ast("1 ** 2") }.to raise_error Lexr::UnmatchableTextError
end
it "should raise an exception when missing a closing parenthesis" do
expect { build_ast("(((123))") }.to raise_error MathEngine::ParseError
end
it "should raise an exception when missing a parameter" do
expect { build_ast("abc(1, )") }.to raise_error MathEngine::ParseError
end
end
<file_sep>class MathEngine
module Evaluators
def self.find_by_name(name)
class_name = name.to_s.sub(%r{^[a-z\d]}) { $&.upcase }
class_name.gsub!(%r{(?:_|(\/))([a-z\d]*)}) { "#{$1}#{$2.capitalize}" }
MathEngine::Evaluators.const_get(class_name) rescue raise MathEngine::UnknownEvaluatorError.new(name, "MathEngine::Evaluators::#{class_name}")
end
end
end<file_sep>require File.expand_path(File.join(File.dirname(__FILE__), '..', 'spec_helper'))
describe "Finding evaluators by name" do
it "should by able to find the calculate evaluator" do
expect(MathEngine::Evaluators.find_by_name(:calculate)).to eq(MathEngine::Evaluators::Calculate)
end
it "should raise an error if unable to find the evaluator" do
expect { MathEngine::Evaluators.find_by_name(:not_there) }.to raise_error MathEngine::UnknownEvaluatorError.new(:not_there, "MathEngine::Evaluators::NotThere")
end
end
<file_sep>class MathEngine
class Node
attr_reader :left, :right
alias :value :left
def initialize(left, right = nil)
@left, @right = left, right
end
def evaluate(evaluator)
evaluator.send(method_name, self)
end
private
def method_name
class_name = self.class.name[0..-5]
class_name = class_name[class_name.rindex("::")+2..-1] if class_name.rindex("::")
method_name = class_name.gsub(%r{([A-Z\d]+)([A-Z][a-z])},'\1_\2').gsub(%r{([a-z\d])([A-Z])},'\1_\2').downcase
end
end
class LiteralNumberNode < Node ; end
class ExpressionNode < Node ; end
class ParenthesisedExpressionNode < Node ; end
class IdentifierNode < Node ; end
class AssignmentNode < Node ; end
class AdditionNode < Node ; end
class SubtractionNode < Node ; end
class MultiplicationNode < Node ; end
class DivisionNode < Node ; end
class ExponentNode < Node ; end
class ModulusNode < Node ; end
class FunctionCallNode < Node ; end
class ParametersNode < Node
def to_a
[left] + (right ? right.to_a : [])
end
end
end<file_sep>require "rubygems"
require "bundler"
Bundler.require :default, :test
require File.expand_path(File.join(File.dirname(__FILE__), '..', 'lib', 'math_engine'))
def evaluate(line)
MathEngine.new.evaluate(line)
end
def build_ast(line)
MathEngine::Parser.new(MathEngine::Lexer.new(line)).parse
end
def print_ast(node, indent = "")
puts "#{node.class.name} #{node.is_a?(MathEngine::Node) ? "" : "(#{node})"}"
return unless node.is_a? MathEngine::Node
print "#{indent}left: " if node.left
print_ast(node.left, indent + " ") if node.left
print "#{indent}right: " if node.right
print_ast(node.right, indent + " ") if node.right
end<file_sep>class MathEngine
class ParseError < StandardError
def initialize(message)
@message = message
end
def to_s
@message
end
end
class UnknownVariableError < StandardError
def initialize(variable_name)
@variable_name = variable_name
end
def to_s
"Variable '#{@variable_name}' was referenced but does not exist"
end
end
class UnknownFunctionError < StandardError
def initialize(function_name)
@function_name = function_name
end
def to_s
"Function '#{@function_name}' was referenced but does not exist"
end
end
class UnableToModifyConstantError < StandardError
def initialize(constant_name)
@constant_name = constant_name
end
def to_s
"Unable to modify value of constant '#{@constant_name}'"
end
end
class UnknownEvaluatorError < StandardError
def initialize(evaluator_name, expected_const)
@evaluator_name = evaluator_name
@expected_const = expected_const
end
def to_s
"Unable to find an evaluator called #{@evaluator_name}(#{@expected_const})"
end
def ==(other)
self.to_s == other.to_s
end
end
end<file_sep>require File.expand_path(File.join(File.dirname(__FILE__), '..', 'spec_helper'))
class RandomlyNamedNode < MathEngine::Node ; end
module AnotherTest
class RandomlyNamedNode < MathEngine::Node ; end
module AndAgain
class RandomlyNamedNode < MathEngine::Node ; end
end
end
class MockEvaluator
attr_reader :call_list
def initialize
@call_list = []
end
def method_missing(name, *args, &block)
@call_list << {name: name, args: args}
end
end
describe "Node evaluation against the evaluator" do
it "should call the correct method on the evaluator based on its own name when not in a module" do
evaluator = MockEvaluator.new
node = RandomlyNamedNode.new(Object.new)
node.evaluate(evaluator)
expect(evaluator.call_list).to eq([{name: :randomly_named, args: [node]}])
end
it "should call the correct method on the evaluator based on its own name when in a module" do
evaluator = MockEvaluator.new
node = AnotherTest::RandomlyNamedNode.new(Object.new)
node.evaluate(evaluator)
expect(evaluator.call_list).to eq([{name: :randomly_named, args: [node]}])
end
it "should call the correct method on the evaluator based on its own name when in a module multiple levels deep" do
evaluator = MockEvaluator.new
node = AnotherTest::AndAgain::RandomlyNamedNode.new(Object.new)
node.evaluate(evaluator)
expect(evaluator.call_list).to eq([{name: :randomly_named, args: [node]}])
end
end
<file_sep>class MathEngine
class Context
DEFAULT_OPTIONS = {case_sensitive: true}
def initialize(opts = {})
@opts = DEFAULT_OPTIONS.merge(opts)
@variables = {}
@dynamic_library = Class.new.new
@libraries = [@dynamic_library, Math]
end
def get(variable_name)
@variables[key variable_name]
end
def set(variable_name, value)
raise MathEngine::UnableToModifyConstantError.new(key variable_name) if constant?(key variable_name) &&
get(variable_name)
@variables[key variable_name] = value
end
def define(function_name, func = nil, &block)
@dynamic_library.class.send :define_method, function_name.to_sym do |*args|
func ? func.call(*args) : block.call(*args)
end
end
def call(function_name, *args)
library = library_for_function(function_name)
raise UnknownFunctionError.new(function_name) unless library
library.send(function_name.to_sym, *args)
end
def include_library(library)
@libraries << library
end
def constants
@variables.keys.select { |variable_name| constant?(variable_name) }
end
def variables
@variables.keys - constants
end
private
def constant?(variable_name)
variable_name.upcase == variable_name
end
def key(variable_name)
return variable_name if @opts[:case_sensitive]
result = @variables.keys.select { |key| key.downcase == variable_name.downcase }.first
result || variable_name
end
def library_for_function(function_name)
@libraries.detect { |l| l.respond_to?(function_name.to_sym) }
end
end
end | 59ec12943499dddb94d4c81cda7a8da8e9c76537 | [
"Markdown",
"Ruby"
] | 18 | Ruby | mikebaldry/math_engine | b2f0be3f37beda16ad4c8216f652da49daf812c5 | 704a9de85236dc8d5416bbccb862121aa9e07e67 | |
refs/heads/master | <repo_name>NikemoXss/AsScg<file_sep>/app/build.gradle
apply plugin: 'com.android.application'
android {
compileSdkVersion 26
buildToolsVersion "25.0.3"
defaultConfig {
applicationId "com.ys.www.asscg"
minSdkVersion 16
targetSdkVersion 24
versionCode 1
versionName "1.0"
testInstrumentationRunner "android.support.test.runner.AndroidJUnitRunner"
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
}
}
sourceSets {
main {
jniLibs.srcDir 'src/main/jniLibs'
}
}
}
dependencies {
compile fileTree(include: ['*.jar'], dir: 'libs')
androidTestCompile('com.android.support.test.espresso:espresso-core:2.2.2', {
exclude group: 'com.android.support', module: 'support-annotations'
})
compile 'com.android.support:appcompat-v7:26.+'
compile 'com.android.support.constraint:constraint-layout:1.0.2'
compile 'com.squareup.okhttp3:okhttp:3.8.1'
compile 'com.android.support:design:26.+'
testCompile 'junit:junit:4.12'
compile 'com.nineoldandroids:library:2.4.0'
compile 'com.nostra13.universalimageloader:universal-image-loader:1.9.5'
compile 'com.google.code.gson:gson:2.8.1'
compile 'com.squareup.picasso:picasso:2.5.2'
compile 'com.android.support:recyclerview-v7:26.0.0-alpha1'
compile files('libs/baidumapapi_base_v4_3_1.jar')
compile files('libs/baidumapapi_cloud_v4_3_1.jar')
compile files('libs/baidumapapi_map_v4_3_1.jar')
compile files('libs/baidumapapi_radar_v4_3_1.jar')
compile files('libs/baidumapapi_search_v4_3_1.jar')
compile files('libs/baidumapapi_util_v4_3_1.jar')
compile files('libs/locSDK_6.13.jar')
compile files('libs/MobCommons-2017.0608.1618.jar')
compile files('libs/MobTools-2017.0608.1618.jar')
compile files('libs/ShareSDK-Core-3.0.0.jar')
compile files('libs/ShareSDK-QQ-3.0.0.jar')
compile files('libs/ShareSDK-QZone-3.0.0.jar')
compile files('libs/ShareSDK-Wechat-3.0.0.jar')
compile files('libs/ShareSDK-Wechat-Core-3.0.0.jar')
compile files('libs/ShareSDK-Wechat-Favorite-3.0.0.jar')
compile files('libs/ShareSDK-Wechat-Moments-3.0.0.jar')
}
<file_sep>/app/src/main/java/com/ys/www/asscg/fragment/SetFragment_Scg.java
package com.ys.www.asscg.fragment;
import android.content.Intent;
import android.os.Bundle;
import android.os.Handler;
import android.os.Message;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.view.View.OnClickListener;
import android.view.ViewGroup;
import com.ys.www.asscg.R;
import com.ys.www.asscg.activity.AboutUsActivity;
import com.ys.www.asscg.activity.ContactUsActivity;
import com.ys.www.asscg.activity.FeedbackActivity;
import com.ys.www.asscg.activity.LoginActivity;
import com.ys.www.asscg.api.SystenmApi;
import com.ys.www.asscg.base.BaseFragment;
import com.ys.www.asscg.http.HttpClient;
import com.ys.www.asscg.util.Default;
import org.json.JSONException;
import org.json.JSONObject;
//苏常设置
public class SetFragment_Scg extends BaseFragment implements OnClickListener {
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
View mainView = inflater.inflate(R.layout.layout_set, null);
mainView.findViewById(R.id.item_feedback).setOnClickListener(this);
mainView.findViewById(R.id.item_check_update).setOnClickListener(this);
mainView.findViewById(R.id.item_shareapp).setOnClickListener(this);
mainView.findViewById(R.id.item_contactus).setOnClickListener(this);
mainView.findViewById(R.id.item_aboutus).setOnClickListener(this);
return mainView;
}
@Override
public void onResume() {
// TODO Auto-generated method stub
super.onResume();
}
public void stop() {
}
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.item_feedback:
// 检测用户是否登录
if (Default.userId != 0) {
getActivity().startActivity(new Intent(getActivity(), FeedbackActivity.class));
} else {
getActivity().startActivity(new Intent(getActivity(), LoginActivity.class));
}
break;
case R.id.item_check_update:
new Thread() {
@Override
public void run() {
super.run();
checkNewVersion();
}
}.start();
break;
case R.id.item_shareapp:
SystenmApi.showShareView(getActivity(), "苏常网APP,随时随地掌握你的财富", "手机移动理财的指尖神器,帮您在“拇指时代”指点钱途,“掌握财富”。",
"http://www.czsuchang.com/Member/Common/AppRegister?invite=" + Default.userId);//http://www.czsuchang.com/Member/Common/AppRegister?invite=881
break;
case R.id.item_contactus:
Intent intent = new Intent(getActivity(), ContactUsActivity.class);
startActivity(intent);
break;
case R.id.item_aboutus:
Intent intent1 = new Intent(getActivity(), AboutUsActivity.class);
startActivity(intent1);
break;
default:
break;
}
}
// 提交用户反馈到服务器
public void checkNewVersion() {
String url = Default.ip + Default.version;
JSONObject jsonObject = new JSONObject();
try {
jsonObject.put("uid", 0);
jsonObject.put("version", Default.version);
} catch (JSONException e) {
e.printStackTrace();
}
try {
String s = HttpClient.post(url, jsonObject.toString());
if (!"".equals(s)) {
JSONObject response = str2json(s);
Message msg = new Message();
msg.what = 1;
msg.obj = response;
handler.sendMessage(msg);
} else {
showCustomToast("获取失败");
}
Log.e("HttpClient", s);
} catch (Exception e) {
e.printStackTrace();
}
}
public JSONObject str2json(String s) {
JSONObject json = null;
try {
json = new JSONObject(s);
} catch (JSONException e) {
e.printStackTrace();
}
return json;
}
Handler handler = new Handler() {
@Override
public void handleMessage(Message msg) {
super.handleMessage(msg);
switch (msg.what) {
case 1:
JSONObject response = (JSONObject) msg.obj;
try {
// 没有新版本
if (response.getInt("status") == 1) {
showCustomToast(response.getString("message"));
// 获取新版本
} else if (response.getInt("status") == 0) {
// 另起后台线程 下载新版APP
} else {
showCustomToast(response.getString("message"));
}
} catch (JSONException e) {
e.printStackTrace();
}
break;
default:
break;
}
}
};
}
<file_sep>/app/src/main/java/com/ys/www/asscg/activity/itemNewsActivity.java
package com.ys.www.asscg.activity;
import android.graphics.drawable.Drawable;
import android.os.Bundle;
import android.os.Handler;
import android.os.Message;
import android.text.Html;
import android.text.Html.ImageGetter;
import android.text.Spanned;
import android.util.Log;
import android.view.View;
import android.view.View.OnClickListener;
import android.webkit.WebSettings.LayoutAlgorithm;
import android.webkit.WebView;
import android.widget.ImageView;
import android.widget.TextView;
import com.ys.www.asscg.R;
import com.ys.www.asscg.base.BaseActivity;
import com.ys.www.asscg.http.HttpClient;
import com.ys.www.asscg.item.newsItem;
import com.ys.www.asscg.util.Default;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import java.io.InputStream;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.HashMap;
import java.util.Map;
public class itemNewsActivity extends BaseActivity implements OnClickListener {
public static String source; // =
private TextView name;
private TextView time;
private newsItem mItem;
private WebView webview;
public static JSONObject noticeListItemJson;
ImageView title_right;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.news);
name = (TextView) findViewById(R.id.newsName);
time = (TextView) findViewById(R.id.newsTime);
title_right= findViewById(R.id.title_right);
title_right.setVisibility(View.VISIBLE);
title_right.setOnClickListener(this);
TextView text = (TextView) findViewById(R.id.title);
text.setText(R.string.newsTitle);
webview = (WebView) findViewById(R.id.web);
webview.getSettings().setLayoutAlgorithm(LayoutAlgorithm.SINGLE_COLUMN);
new Thread() {
@Override
public void run() {
super.run();
if (Default.IS_SHOW_NESW_OR_NOTICE) {
doShowItemhttp(Default.showNoticeId);
} else {
doShowItemhttp(Default.showNewsId);
}
}
}.start();
}
private String getFormateHtml(String html) {
String retStr = "<html><head><style type='text/css'>p{text-align:justify;border-style:" + " none;border-top-width: 2px;border-right-width: 2px;border-bottom-width: 2px;border-left-width: 2px;}" + "img{height:auto;width: auto;width:100%;}</style></head><body>" + html + "</body></html>";
return retStr;
}
private void updateData(JSONObject json) {
try {
name.setText(json.getString("title"));
time.setText(json.getString("art_time"));
source = json.getString("art_content");
// doText();
webview.loadDataWithBaseURL(Default.ip, getFormateHtml(source), "text/html", "utf-8", null);
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.title_right:
finish(0);
break;
}
}
public void doText() {
new Thread(new Runnable() {
@Override
public void run() {
ImageGetter getter = new ImageGetter() {
@Override
public Drawable getDrawable(String source) {
Drawable drawable = null;
try {
URL url = new URL(Default.ip + source);
String srcName = source.substring(source.lastIndexOf("/") + 1, source.length());
InputStream is = null;
try {
is = url.openStream();
} catch (IOException e) {
e.printStackTrace();
}
drawable = Drawable.createFromStream(is, srcName);
drawable.setBounds(0, 0, drawable.getIntrinsicWidth(), drawable.getIntrinsicHeight());
} catch (MalformedURLException e) {
e.printStackTrace();
}
return drawable;
}
};
Spanned spanned = Html.fromHtml(source, getter, null);
Map map = new HashMap<String, Object>();
map.put("spanned", spanned);
map.put("info", source);
Message msg = new Message();
msg.what = 1;
msg.obj = map;
handler.sendMessage(msg);
}
}).start();
}
@Override
public void finish() {
Default.IS_SHOW_NESW_OR_NOTICE = false;
super.finish();
}
Handler handler = new Handler() {
@Override
public void handleMessage(Message msg) {
switch (msg.what) {
case 1:
HashMap map = (HashMap) msg.obj;
Spanned getter = (Spanned) map.get("spanned");
// info.setText(getter);
break;
case 3:
updateData(noticeListItemJson);
break;
}
}
};
// private void doShowItemhttp(long id)
// {
// showLoadingDialogNoCancle(getResources().getString(R.string.toast2));
//
// JsonBuilder builder = new JsonBuilder();
// builder.put("id", id);
// new BaseModel(null, Default.newsListItem, builder)
// .setConnectionResponseLinstener(new ConnectResponseListener()
// {
// public void onConnectResponseCallback(JSONObject json)
// {
// dismissLoadingDialog();
// Data.noticeListItemJson = json;
// handler.sendEmptyMessage(3);
// }
//
// @Override
// public void onFail(JSONObject json)
// {
// dismissLoadingDialog();
// try
// {
// Message msg = new Message();
// msg.arg1 = 2;
// Bundle bundel = new Bundle();
// bundel.putString("info", json.getString("message"));
// msg.setData(bundel);
// handler.sendMessage(msg);
// }
// catch (JSONException e)
// {
// e.printStackTrace();
// }
// }
// });
//
// }
private void doShowItemhttp(long id) {
String url = Default.ip + (Default.IS_SHOW_NESW_OR_NOTICE ? Default.noticeListItem : Default.newsListItem);
JSONObject jsonObject = new JSONObject();
try {
jsonObject.put("uid", 0);
jsonObject.put("id", id);
} catch (JSONException e) {
e.printStackTrace();
}
try {
String s = HttpClient.post(url, jsonObject.toString());
if (!"".equals(s)) {
JSONObject json = str2json(s);
noticeListItemJson = json;
handler.sendEmptyMessage(3);
} else {
showCustomToast("获取失败");
}
Log.e("HttpClient", s);
} catch (IOException e) {
e.printStackTrace();
}
}
public JSONObject str2json(String s) {
JSONObject json = null;
try {
json = new JSONObject(s);
} catch (JSONException e) {
e.printStackTrace();
}
return json;
}
}
<file_sep>/app/src/main/java/com/ys/www/asscg/activity/ForgotPwdActivity.java
package com.ys.www.asscg.activity;
import android.content.Intent;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.EditText;
import android.widget.ImageView;
import android.widget.TextView;
import com.ys.www.asscg.R;
import com.ys.www.asscg.base.BaseActivity;
import com.ys.www.asscg.http.HttpClient;
import com.ys.www.asscg.util.Default;
import org.json.JSONException;
import org.json.JSONObject;
public class ForgotPwdActivity extends BaseActivity implements OnClickListener {
/**
* 用户手机号码
*/
private EditText phoneEditor;
private EditText usernameEditor, user_phone_sx, ver_pwd_sx;
/**
* 用户手机号码
*/
private String phoneNum;
private String username;
TextView title;
ImageView iv;
String uid;
String iszfpwd;
public static ForgotPwdActivity instance = null;
@Override
protected void onCreate(Bundle savedInstanceState) {
// TODO Auto-generated method stub
super.onCreate(savedInstanceState);
setContentView(R.layout.forgot_pwd);
instance = this;
title = (TextView) findViewById(R.id.title);
Intent intent = getIntent();
iszfpwd = intent.getStringExtra("iszfpwd");
// showCustomToast(iszfpwd);
title.setText("忘记登录密码");
if(iszfpwd.equals("0")){
title.setText("忘记支付密码");
}
// findViewById(R.id.forget_pwd).setOnClickListener(this);
iv = (ImageView) findViewById(R.id.title_right);
iv.setVisibility(View.VISIBLE);
iv.setOnClickListener(this);
// phoneEditor = (EditText) findViewById(R.id.user_phone);
// usernameEditor = (EditText) findViewById(R.id.user_name);
findViewById(R.id.edittext_sx).setOnClickListener(this);
user_phone_sx = (EditText) findViewById(R.id.user_phone_sx);
findViewById(R.id.forget_pwd_sx).setOnClickListener(this);
ver_pwd_sx = (EditText) findViewById(R.id.ver_pwd_sx);
}
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
switch (v.getId()) {
case R.id.title_right:
finish();
break;
case R.id.forget_pwd_sx:// 校验验证码
if (user_phone_sx.equals("")) {
showCustomToast("请输入您的手机号!");
return;
}
if (ver_pwd_sx.equals("")) {
showCustomToast("请输入验证码!");
return;
}
doHttpyzmyz();
// Intent intent = new Intent(ForgotPwdActivity.this, AddNewPwd.class);
// intent.putExtra("uid", 349);
// startActivity(intent);
break;
case R.id.edittext_sx:// 获取验证码
doHttpyzm();
break;
default:
break;
}
}
private void doHttpyzm() {
{
String url = Default.ip + Default.getyzm;
try {
JSONObject jsonObject=new JSONObject();
jsonObject.put("uid", 0);
jsonObject.put("phone", user_phone_sx.getText().toString());
String s = HttpClient.post(url, jsonObject.toString());
if (!"".equals(s)) {
JSONObject response = str2json(s);
if (response.getInt("status") == 1) {
uid = response.getString("uid");
showCustomToast("验证码发送成功");
} else {
showCustomToast("验证码发送失败");
}
} else {
showCustomToast("获取失败");
}
Log.e("HttpClient", s);
} catch (Exception e) {
e.printStackTrace();
}
}
}
public JSONObject str2json(String s) {
JSONObject json = null;
try {
json = new JSONObject(s);
} catch (JSONException e) {
e.printStackTrace();
}
return json;
}
private void doHttpyzmyz() {
String url = Default.ip + Default.yzmyzsx;
try {
JSONObject jsonObject=new JSONObject();
jsonObject.put("uid", 0);
jsonObject.put("code", ver_pwd_sx.getText().toString());
String s = HttpClient.post(url, jsonObject.toString());
if (!"".equals(s)) {
JSONObject response = str2json(s);
if (response.getInt("status") == 1) {
Intent intent = new Intent(ForgotPwdActivity.this, AddNewPwd.class);
intent.putExtra("uid", uid);
intent.putExtra("iszf", iszfpwd);
startActivity(intent);
} else {
showCustomToast("验证码发送失败");
}
} else {
showCustomToast("验证码验证错误");
}
Log.e("HttpClient", s);
} catch (Exception e) {
e.printStackTrace();
}
}
@Override
public void finish() {
// TODO Auto-generated method stub
super.finish();
}
@Override
protected void onDestroy() {
// TODO Auto-generated method stub
super.onDestroy();
}
}
<file_sep>/app/src/main/java/com/ys/www/asscg/activity/VIPActivity.java
package com.ys.www.asscg.activity;
import android.content.Intent;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.AdapterView;
import android.widget.AdapterView.OnItemClickListener;
import android.widget.EditText;
import android.widget.ImageView;
import android.widget.TextView;
import android.widget.Toast;
import com.ys.www.asscg.R;
import com.ys.www.asscg.api.SystenmApi;
import com.ys.www.asscg.base.BaseActivity;
import com.ys.www.asscg.dialog.PopDialog;
import com.ys.www.asscg.http.HttpClient;
import com.ys.www.asscg.util.Default;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
public class VIPActivity extends BaseActivity implements OnClickListener {
private TextView vip_free_textView;
private EditText vip_info_editText;
private JSONArray kf_list;
private String kf_id;
private PopDialog kf_popDialog;
private TextView user_select_kfName, title;
ImageView imageView;
private boolean isClickItem = false;
private int index = 0;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.vip);
initView();
}
public void initView() {
imageView = (ImageView) findViewById(R.id.title_right);
imageView.setVisibility(View.VISIBLE);
imageView.setOnClickListener(this);
title = (TextView) findViewById(R.id.title);
title.setText("VIP申请");
findViewById(R.id.vip_button).setOnClickListener(this);
vip_free_textView = (TextView) findViewById(R.id.vip_fee);
kf_popDialog = new PopDialog(VIPActivity.this);
kf_popDialog.setDialogTitle("选择客服");
user_select_kfName = (TextView) findViewById(R.id.show_selecet_name);
findViewById(R.id.vip_kf).setOnClickListener(this);
kf_popDialog.setonClickListener(this);
kf_popDialog.setOnItemClickListener(new OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
isClickItem = true;
kf_popDialog.setDefaultSelect(position);
index = position;
}
});
vip_info_editText = (EditText) findViewById(R.id.vip_info);
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.title_right:
finish(0);
break;
case R.id.vip_kf:
if (kf_list != null) {
SystenmApi.hiddenKeyBoard(VIPActivity.this);
kf_popDialog.setDefaultSelect(0);
try {
kf_id = kf_list.getJSONObject(0).getString("id");
} catch (JSONException e) {
e.printStackTrace();
}
kf_popDialog.showAsDropDown(v);
}
break;
case R.id.cButton:
kf_popDialog.dismiss();
break;
case R.id.sButton:
if (isClickItem) {
try {
kf_id = kf_list.getJSONObject(index).getString("id");
user_select_kfName
.setText(kf_list.getJSONObject(kf_popDialog.getDefaultSelect()).getString("user_name"));
} catch (JSONException e) {
e.printStackTrace();
}
} else {
kf_popDialog.setDefaultSelect(0);
try {
user_select_kfName.setText(kf_list.getJSONObject(0).getString("user_name"));
kf_id = kf_list.getJSONObject(0).getString("id");
} catch (JSONException e) {
e.printStackTrace();
}
}
kf_popDialog.dismiss();
break;
case R.id.vip_button:
if (kf_id == null) {
showCustomToast("请选择客服");
return;
} else if (vip_info_editText.getText().toString().equals("")) {
showCustomToast("请输入申请说明");
return;
} else {
new Thread() {
@Override
public void run() {
super.run();
vipActiondohttp();
}
}.start();
}
break;
}
}
private void initInfo(JSONObject json) {
try {
vip_free_textView.setText(json.getString("fee_vip") + "元/年");
kf_list = json.getJSONArray("list");
for (int i = 0; i < kf_list.length(); i++) {
JSONObject obj = kf_list.getJSONObject(i);
kf_popDialog.addItem(obj.getString("user_name"));
}
} catch (Exception e) {
e.printStackTrace();
}
}
@Override
protected void onResume() {
// TODO Auto-generated method stub
super.onResume();
new Thread() {
@Override
public void run() {
super.run();
doHttp();
}
}.start();
}
private void doHttp() {
String url = Default.ip + Default.vip_kf_inf;
try {
String s = HttpClient.post(url, null);
if (!"".equals(s)) {
JSONObject response = str2json(s);
if (response.has("status")) {
try {
if (response.getInt("status") == 1) {
initInfo(response);
}
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
} else {
showCustomToast("获取失败");
}
Log.e("HttpClient", s);
} catch (Exception e) {
e.printStackTrace();
}
}
private void vipActiondohttp() {
String url = Default.ip + Default.hf_vip;
try {
String s = HttpClient.post(url, null);
if (!"".equals(s)) {
JSONObject response = str2json(s);
if (response.has("status")) {
try {
if (response.getInt("status") == 1) {
showCustomToast("提交申请成功,请等待管理员审核");
} else {
showCustomToast(response.getString("message"));
}
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
} else {
showCustomToast("获取失败");
}
Log.e("HttpClient", s);
} catch (Exception e) {
e.printStackTrace();
}
}
public JSONObject str2json(String s) {
JSONObject json = null;
try {
json = new JSONObject(s);
} catch (JSONException e) {
e.printStackTrace();
}
return json;
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
int code = data.getIntExtra("code", -1);
String message = data.getStringExtra("message");
int code1 = data.getIntExtra("code1", -1);
String message1 = data.getStringExtra("message1");
int code2 = data.getIntExtra("code2", -1);
String message2 = data.getStringExtra("message2");
if (code == 88) {
Toast.makeText(this, "恭喜您,支付成功!", Toast.LENGTH_SHORT).show();
finish();
} else {
Toast.makeText(this, code + ":" + message, Toast.LENGTH_SHORT).show();
}
}
@Override
public void finish() {
super.finish();
}
}
<file_sep>/app/src/main/java/com/ys/www/asscg/adapter/MyReViewAdapter.java
package com.ys.www.asscg.adapter;
import android.content.Context;
import android.content.Intent;
import android.support.v7.widget.RecyclerView;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.FrameLayout;
import android.widget.ImageView;
import android.widget.ProgressBar;
import android.widget.TextView;
import com.ys.www.asscg.R;
import com.ys.www.asscg.activity.BidItem;
import java.util.List;
import java.util.Map;
/**
* Created by Administrator on 2017/6/23.
*/
public class MyReViewAdapter extends RecyclerView.Adapter<MyReViewAdapter.ViewHolder> {
List<Map<String, Object>> list;
Context context;
private LayoutInflater mInflater;
public MyReViewAdapter(List<Map<String, Object>> list, Context context) {
this.list = list;
this.context = context;
mInflater = LayoutInflater.from(context);
}
@Override
public ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View view = mInflater.inflate(R.layout.item_list_indexfragment, parent, false);
ViewHolder myViewHolder = new ViewHolder(view);
return myViewHolder;
}
@Override
public void onBindViewHolder(ViewHolder holder,final int position) {
if (!"1".equals(list.get(position).get("repayment_type").toString())) {// 1表示天标
// 其余数值表示月标
holder.title_icon_r.setBackgroundResource(R.drawable.image_r2);
holder.title_icon_l.setBackgroundResource(R.drawable.title_i2);
holder.item_bg.setBackgroundResource(R.drawable.item_bg_2);
} else {
holder.title_icon_r.setBackgroundResource(R.drawable.image_r1);
holder.title_icon_l.setBackgroundResource(R.drawable.title_i1);
holder.item_bg.setBackgroundResource(R.drawable.item_bg_1);
}
holder.list_progress.setProgress((int) Double.parseDouble(list.get(position).get("progress_tz_sx").toString()));
holder.list_borrow_name.setText(list.get(position).get("item_tzbt_sx").toString());
holder.list_borrow_money.setText(list.get(position).get("item_jkje_sx").toString());
holder.list_has_borrow.setText(list.get(position).get("has_borrow_sx").toString());
holder.list_borrow_duration.setText(list.get(position).get("item_tzqx_sx").toString());
holder.list_borrow_interest_rate.setText(list.get(position).get("item_nhl_sx").toString());
holder.list_jindu.setText("进度:" + list.get(position).get("progress_tz_sx").toString() + "%");
holder.item_bg.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
Long id = (Long) list.get(position).get("id");
int type = (Integer) list.get(position).get("type");
String string = (String) list.get(position).get("repayment_type");
// Toast.makeText(context, "hahaha"+id, Toast.LENGTH_SHORT).show();
Intent intent = new Intent(context, BidItem.class);
intent.putExtra("id", id);
intent.putExtra("type", type);
context.startActivity(intent);
}
});
}
@Override
public int getItemCount() {
return list.size();
}
public static class ViewHolder extends RecyclerView.ViewHolder {
TextView list_borrow_name, list_borrow_money, list_has_borrow, list_borrow_duration, list_borrow_interest_rate,
list_jindu;
ProgressBar list_progress;
ImageView title_icon_r, title_icon_l;
FrameLayout item_bg;
public ViewHolder(View itemView) {
super(itemView);
list_borrow_name = (TextView) itemView.findViewById(R.id.list_borrow_name);
list_borrow_money = (TextView) itemView.findViewById(R.id.list_borrow_money);
list_has_borrow = (TextView) itemView.findViewById(R.id.list_has_borrow);
list_borrow_duration = (TextView) itemView.findViewById(R.id.list_borrow_duration);
list_borrow_interest_rate = (TextView) itemView.findViewById(R.id.list_borrow_interest_rate);
list_jindu = (TextView) itemView.findViewById(R.id.list_jindu);
list_progress = (ProgressBar) itemView.findViewById(R.id.list_progress);
title_icon_r = (ImageView) itemView.findViewById(R.id.title_icon_r);
title_icon_l = (ImageView) itemView.findViewById(R.id.title_icon_l);
item_bg = (FrameLayout) itemView.findViewById(R.id.item_bg);
}
}
}
<file_sep>/app/src/main/java/com/ys/www/asscg/activity/PasswordJaoYiActivity.java
package com.ys.www.asscg.activity;
import android.content.Intent;
import android.os.Bundle;
import android.os.Handler;
import android.os.Message;
import android.util.Log;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.EditText;
import android.widget.ImageView;
import android.widget.TextView;
import com.ys.www.asscg.R;
import com.ys.www.asscg.base.BaseActivity;
import com.ys.www.asscg.http.HttpClient;
import com.ys.www.asscg.util.Default;
import org.json.JSONException;
import org.json.JSONObject;
/**
* 修改交易密码
*/
public class PasswordJaoYiActivity extends BaseActivity implements OnClickListener {
private EditText mOldd, mPassww, mPassww2;
private String info[];
ImageView iv_back;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.people_info_update_jiaoyipsw);
initView();
}
public void initView() {
iv_back = (ImageView) findViewById(R.id.title_right);
iv_back.setVisibility(View.VISIBLE);
iv_back.setOnClickListener(this);
// findViewById(R.id.back).setOnClickListener(this);
findViewById(R.id.btn_jiayoyi).setOnClickListener(this);
findViewById(R.id.getPw).setOnClickListener(this);
TextView text = (TextView) findViewById(R.id.title);
text.setText(R.string.updatezfpsd_title);
mOldd = (EditText) findViewById(R.id.ed_itold);
mPassww = (EditText) findViewById(R.id.ed_itpassw);
mPassww2 = (EditText) findViewById(R.id.ed_itpassw2);
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.btn_jiayoyi:
info = getInfo();
if (info == null) {
return;
}
doHttp();
break;
case R.id.title_right:
finish();
break;
case R.id.getPw:
Intent intent = new Intent(PasswordJaoYiActivity.this, ForgotPwdActivity.class);
intent.putExtra("iszfpwd", "0");
startActivity(intent);
break;
default:
break;
}
}
public String[] getInfo() {
String old = mOldd.getText().toString();
String passw = mPassww.getText().toString();
String passw2 = mPassww2.getText().toString();
if (old.equals("")) {
showCustomToast(R.string.toast7);
return null;
}
if (passw.equals("")) {
showCustomToast(R.string.toast8);
return null;
}
if (passw.length() < 6) {
showCustomToast(R.string.toast8_2);
return null;
}
if (passw.length() > 16) {
showCustomToast(R.string.toast8_3);
return null;
}
if (passw2.equals("")) {
showCustomToast(R.string.toast8_1);
return null;
}
if (passw2.equals(passw) == false) {
showCustomToast(R.string.toast9);
return null;
}
return new String[] { old, passw, passw2 };
}
public void doHttp() {
String url = Default.ip + Default.peoInfoxsjiaoyipsw;
try {
JSONObject jsonObject=new JSONObject();
jsonObject.put("uid", 0);
jsonObject.put("oldpwd", info[0]);
jsonObject.put("newpwd", info[1]);
String s = HttpClient.post(url, jsonObject.toString());
if (!"".equals(s)) {
JSONObject response = str2json(s);
if (response.getInt("status") == 1) {
finish();
showCustomToast("修改支付密码成功!");
} else {
showCustomToast(response.getString("message"));
}
} else {
showCustomToast("获取失败");
}
Log.e("HttpClient", s);
} catch (Exception e) {
e.printStackTrace();
}
}
public JSONObject str2json(String s) {
JSONObject json = null;
try {
json = new JSONObject(s);
} catch (JSONException e) {
e.printStackTrace();
}
return json;
}
private Handler handler = new Handler() {
@Override
public void handleMessage(Message msg) {
super.handleMessage(msg);
showCustomToast(msg.getData().getString("info"));
}
};
}
<file_sep>/app/src/main/java/com/ys/www/asscg/fragment/IndexFragment_Scg.java
package com.ys.www.asscg.fragment;
import android.content.Context;
import android.content.Intent;
import android.graphics.Bitmap;
import android.os.Bundle;
import android.os.Handler;
import android.os.Message;
import android.os.SystemClock;
import android.support.v4.view.ViewPager;
import android.support.v4.view.ViewPager.OnPageChangeListener;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.view.View.OnClickListener;
import android.view.ViewGroup;
import android.widget.ImageView;
import android.widget.ImageView.ScaleType;
import android.widget.LinearLayout;
import android.widget.ProgressBar;
import android.widget.TextView;
import com.nostra13.universalimageloader.core.DisplayImageOptions;
import com.nostra13.universalimageloader.core.ImageLoader;
import com.nostra13.universalimageloader.core.assist.FailReason;
import com.nostra13.universalimageloader.core.assist.ImageScaleType;
import com.nostra13.universalimageloader.core.display.FadeInBitmapDisplayer;
import com.nostra13.universalimageloader.core.listener.SimpleImageLoadingListener;
import com.ys.www.asscg.R;
import com.ys.www.asscg.activity.BidItem;
import com.ys.www.asscg.activity.LoginActivity;
import com.ys.www.asscg.activity.NewsListActivity;
import com.ys.www.asscg.activity.TouziActivity;
import com.ys.www.asscg.base.BaseFragment;
import com.ys.www.asscg.http.HttpClient;
import com.ys.www.asscg.util.Default;
import com.ys.www.asscg.view.AutoVerticalScrollTextView;
import com.ys.www.asscg.view.ViewPagerAdapter;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.List;
import static com.ys.www.asscg.activity.MainTabActivity.mainTabActivity;
//苏常主页
public class IndexFragment_Scg extends BaseFragment implements OnClickListener, OnPageChangeListener {
private int curPage = 1, pageCount = 1;
private JSONArray list = null;
private long id_tt, id_yy;
private int type_tt, type_yy;
private ViewPagerAdapter vpAdapter;
private List<View> views;
private ArrayList<View> footPointViews;
private List<String> bannerItemspic;
private LinearLayout footpointview;
private static final int[] pics = {R.drawable.slide};
private ViewPager flipper;
private int index;
private boolean change;
private int number = 0;
private boolean isRunning = true;
LinearLayout item_tt, item_yy;
private AutoVerticalScrollTextView autoVerticalScrollTextView;
TextView tt_borrow_name, tt_borrow_money, tt_has_borrow, tt_borrow_duration, tt_borrow_interest_rate, tt_jindu;
ProgressBar tt_progress;
TextView yy_borrow_name, yy_borrow_money, yy_has_borrow, yy_borrow_duration, yy_borrow_interest_rate, yy_jindu;
ProgressBar yy_progress;
List<String> list_str;
List<String> mStr;
private String[] strings1 = {"我的剑,就是你的剑!", "俺也是从石头里蹦出来得!", "我用双手成就你的梦想!", "人在塔在!", "犯我德邦者,虽远必诛!", "我会让你看看什么叫残忍!",
"我的大刀早已饥渴难耐了!"};
private Handler mHandler = new Handler();
private Runnable runable = new Runnable() {
@Override
public void run() {
changePic();
}
};
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
View mainView = inflater.inflate(R.layout.layout_index, null);
mainView.findViewById(R.id.gg_loadmore).setOnClickListener(this);
footpointview = (LinearLayout) mainView.findViewById(R.id.footer_point);
autoVerticalScrollTextView = (AutoVerticalScrollTextView) mainView
.findViewById(R.id.autoVerticalScrollTextView);
// autoVerticalScrollTextView.setText(strings[0]);
autoVerticalScrollTextView.setOnClickListener(this);
mainView.findViewById(R.id.item_yy).setOnClickListener(this);
mainView.findViewById(R.id.item_tt).setOnClickListener(this);
initView(mainView);
initTtview(mainView);
initYyview(mainView);
mHandler.postDelayed(runable, 5000);
return mainView;
}
public void initBannerData(JSONObject json) {
if (json != null) {
views.clear();
// flipper.removeAllViews();
flipper.setBackgroundDrawable(null);
footPointViews.clear();
footpointview.removeAllViews();
bannerItemspic = new ArrayList<String>();
try {
JSONArray array = json.getJSONArray("list");
for (int i = 0; i < array.length(); i++) {
JSONObject jsonObject = array.getJSONObject(i);
bannerItemspic.add(jsonObject.get("pic").toString());
// bannerItemspic.add(array.getString(i));
}
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
for (int i = 0; i < bannerItemspic.size(); i++) {
ImageView footView = new ImageView(mainTabActivity);
LinearLayout.LayoutParams params = new LinearLayout.LayoutParams(
ViewGroup.LayoutParams.WRAP_CONTENT,
ViewGroup.LayoutParams.WRAP_CONTENT);
params.leftMargin = 10;
footView.setLayoutParams(params);
if (i == 0) {
footView.setImageResource(R.drawable.b_dx1);
} else {
footView.setImageResource(R.drawable.b_dx2);
}
footpointview.addView(footView);
footPointViews.add(footView);
final ImageView iv = new ImageView(mainTabActivity);
iv.setScaleType(ScaleType.FIT_XY);
iv.setTag(i);
DisplayImageOptions options = new DisplayImageOptions.Builder().showImageForEmptyUri(pics[0])
.showImageOnFail(pics[0]).resetViewBeforeLoading(true).cacheOnDisk(true)
.imageScaleType(ImageScaleType.EXACTLY).bitmapConfig(Bitmap.Config.RGB_565)
.considerExifParams(true).displayer(new FadeInBitmapDisplayer(300)).build();
Log.e("url", bannerItemspic.get(i));
ImageLoader.getInstance().displayImage(Default.ip + bannerItemspic.get(i), iv, options,
new SimpleImageLoadingListener() {
@Override
public void onLoadingStarted(String imageUri, View view) {
}
@Override
public void onLoadingFailed(String imageUri, View view, FailReason failReason) {
String message = null;
switch (failReason.getType()) {
case IO_ERROR:
message = "Input/Output error";
break;
case DECODING_ERROR:
message = "解析错误";
break;
case NETWORK_DENIED:
message = "网络错误";
break;
case OUT_OF_MEMORY:
message = "内存错误";
break;
case UNKNOWN:
message = "位置错误";
break;
}
}
@Override
public void onLoadingComplete(String imageUri, View view, Bitmap loadedImage) {
if (loadedImage != null) {
iv.setImageBitmap(loadedImage);
}
}
});
views.add(iv);
}
}
}
@Override
public void onResume() {
// TODO Auto-generated method stub
super.onResume();
new Thread() {
@Override
public void run() {
super.run();
doHttp();
}
}.start();
new Thread() {
@Override
public void run() {
super.run();
indexdoHttp();
}
}.start();
new Thread() {
@Override
public void run() {
super.run();
ggdoHttp();
}
}.start();
}
/**
* 首页推荐标
*/
public void indexdoHttp() {
String url = Default.ip + Default.tzList;
JSONObject jsonObject = new JSONObject();
try {
jsonObject.put("uid", 0);
jsonObject.put("is_tuijian", 1);
jsonObject.put("type", 11);
jsonObject.put("page", curPage);
jsonObject.put("limit", pageCount);
} catch (JSONException e) {
e.printStackTrace();
}
try {
String s = HttpClient.post(url, jsonObject.toString());
if (!"".equals(s)) {
updateAddInfo(str2json(s));
} else {
showCustomToast("获取失败");
}
Log.e("HttpClient", s);
} catch (IOException e) {
e.printStackTrace();
}
}
public JSONObject str2json(String s) {
JSONObject json = null;
try {
json = new JSONObject(s);
} catch (JSONException e) {
e.printStackTrace();
}
return json;
}
public void ggdoHttp() {
String url = Default.ip + Default.sygg;
try {
String s = HttpClient.get(url);
if (!"".equals(s)) {
JSONObject json = str2json(s);
if (json.getInt("status") == 1) {
String string = json.getString("msg");
// int count = string.length();
JSONArray jsonArray = json.getJSONArray("msg");
list_str = new ArrayList<String>();
for (int i = 0; i < jsonArray.length(); i++) {
JSONObject jsonObject = jsonArray.getJSONObject(i);
list_str.add(jsonObject.optString("title"));
}
Message message = new Message();
message.obj = list_str;
message.what = 111;
handler.sendMessage(message);
} else {
showCustomToast(json.getString("message"));
}
} else {
showCustomToast("获取失败");
}
Log.e("HttpClient", s);
} catch (Exception e) {
e.printStackTrace();
}
}
public void updateAddInfo(JSONObject json) {
try {
if (!json.isNull("list")) {
list = json.getJSONArray("list");
Message message = new Message();
message.what = 100;
message.obj = list;
handler.sendMessage(message);
}
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
/**
* 获取幻灯片
*/
public void doHttp() {
String url = Default.ip + Default.bannerPic;
JSONObject jsonObject = new JSONObject();
try {
jsonObject.put("uid", 0);
} catch (JSONException e) {
e.printStackTrace();
}
try {
String s = HttpClient.post(url, jsonObject.toString());
if (!"".equals(s)) {
Message message = new Message();
message.what = 666;
message.obj = str2json(s);
handler.sendMessage(message);
} else {
showCustomToast("获取失败");
}
Log.e("HttpClient", s);
} catch (IOException e) {
e.printStackTrace();
}
}
public void stop() {
try {
mHandler.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
public void changePic() {
if (getActivity() == null) {
return;
}
if (!change) {
if (index < views.size() - 1) {
index++;
setImage(index);
flipper.setCurrentItem(index);
if (index == views.size() - 1) {
change = true;
}
}
} else {
if (index > 0) {
index--;
setImage(index);
flipper.setCurrentItem(index);
if (index == 0) {
change = false;
}
}
}
mHandler.postDelayed(runable, 5000);
}
void setImage(int i) {
for (int j = 0; j < footPointViews.size(); j++) {
ImageView iv = (ImageView) footPointViews.get(j);
if (j != i) {
iv.setImageResource(R.drawable.b_dx2);
} else {
iv.setImageResource(R.drawable.b_dx1);
}
}
}
public int px2dip(Context context, float pxValue) {
final float scale = context.getResources().getDisplayMetrics().density;
return (int) (pxValue / scale + 0.5f);
}
public void initView(View view) {
views = new ArrayList<View>();
footPointViews = new ArrayList<View>();
flipper = (ViewPager) view.findViewById(R.id.flipper);
ImageView footView = new ImageView(getActivity());
LinearLayout.LayoutParams params = new LinearLayout.LayoutParams(
ViewGroup.LayoutParams.WRAP_CONTENT, ViewGroup.LayoutParams.WRAP_CONTENT);
params.leftMargin = 10;
footView.setLayoutParams(params);
footView.setImageResource(R.drawable.b_dx1);
footPointViews.add(footView);
footpointview.addView(footView);
flipper = (ViewPager) view.findViewById(R.id.flipper);
vpAdapter = new ViewPagerAdapter(views);
flipper.setAdapter(vpAdapter);
// 绑定回调
flipper.setOnPageChangeListener(this);
// new Thread() {
// @Override
// public void run() {
// while (isRunning) {
// SystemClock.sleep(3000);
// handler.sendEmptyMessage(199);
// }
// }
// }.start();
}
private void initTtview(View mainView) {
// TODO Auto-generated method stub
tt_borrow_name = (TextView) mainView.findViewById(R.id.tt_borrow_name);
tt_borrow_money = (TextView) mainView.findViewById(R.id.tt_borrow_money);
tt_has_borrow = (TextView) mainView.findViewById(R.id.tt_has_borrow);
tt_borrow_duration = (TextView) mainView.findViewById(R.id.tt_borrow_duration);
tt_borrow_interest_rate = (TextView) mainView.findViewById(R.id.tt_borrow_interest_rate);
tt_jindu = (TextView) mainView.findViewById(R.id.tt_jindu);
tt_progress = (ProgressBar) mainView.findViewById(R.id.tt_progress);
}
private void initYyview(View mainView) {
// TODO Auto-generated method stub
yy_borrow_name = (TextView) mainView.findViewById(R.id.yy_borrow_name);
yy_borrow_money = (TextView) mainView.findViewById(R.id.yy_borrow_money);
yy_has_borrow = (TextView) mainView.findViewById(R.id.yy_has_borrow);
yy_borrow_duration = (TextView) mainView.findViewById(R.id.yy_borrow_duration);
yy_borrow_interest_rate = (TextView) mainView.findViewById(R.id.yy_borrow_interest_rate);
yy_jindu = (TextView) mainView.findViewById(R.id.yy_jindu);
yy_progress = (ProgressBar) mainView.findViewById(R.id.yy_progress);
}
private Handler handler = new Handler() {
@SuppressWarnings("unchecked")
@Override
public void handleMessage(Message msg) {
if (msg.what == 199) {
autoVerticalScrollTextView.next();
number++;
// autoVerticalScrollTextView.setText(strings[number %
// strings.length]);
autoVerticalScrollTextView.setText(mStr.get(number % mStr.size()));
} else if (msg.what == 100) {
try {
JSONArray ja = (JSONArray) msg.obj;
JSONObject jo = null;
for (int i = 0; i < ja.length(); i++) {
jo = ja.getJSONObject(i);
if (i == 0) {
id_tt = jo.getLong("id");
type_tt = jo.getInt("borrow_type");
tt_borrow_name.setText(jo.getString("borrow_name"));
tt_borrow_money.setText(jo.getString("borrow_money"));
if ("1".equals(jo.getString("repayment_type"))) {
tt_borrow_duration.setText(jo.getString("borrow_duration") + "天");
} else {
tt_borrow_duration.setText(jo.getString("borrow_duration") + "月");
}
tt_borrow_interest_rate.setText(jo.getString("borrow_interest_rate") + "%");
Double all = jo.getDouble("borrow_money");// getSubtract
Double has = jo.getDouble("has_borrow");
tt_has_borrow.setText(getSubtract(all, has));
int progress = getProgress(all, has);
tt_progress.setProgress(progress);
tt_jindu.setText("进度:" + progress + "%");
} else if (i == 1) {
id_yy = jo.getLong("id");
type_yy = jo.getInt("borrow_type");
yy_borrow_name.setText(jo.getString("borrow_name"));
yy_borrow_money.setText(jo.getString("borrow_money"));
yy_has_borrow.setText(jo.getString("has_borrow"));
if ("1".equals(jo.getString("repayment_type"))) {
yy_borrow_duration.setText(jo.getString("borrow_duration") + "天");
} else {
yy_borrow_duration.setText(jo.getString("borrow_duration") + "月");
}
yy_borrow_interest_rate.setText(jo.getString("borrow_interest_rate") + "%");
Double all = jo.getDouble("borrow_money");
Double has = jo.getDouble("has_borrow");
yy_has_borrow.setText(getSubtract(all, has));
int progress = getProgress(all, has);
yy_progress.setProgress(progress);
yy_jindu.setText("进度:" + progress + "%");
}
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
} else if (msg.what == 111) {
mStr = (List<String>) msg.obj;
if (mStr.size() > 0) {
autoVerticalScrollTextView.setText(mStr.get(0));
new Thread() {
@Override
public void run() {
while (isRunning) {
SystemClock.sleep(3000);
handler.sendEmptyMessage(199);
}
}
}.start();
}
} else if (msg.what == 666) {
JSONObject json = (JSONObject) msg.obj;
initBannerData(json);
vpAdapter.notifyDataSetChanged();
}
}
};
private int getProgress(Double all, Double has) {
BigDecimal b1 = new BigDecimal(all.toString());
BigDecimal b2 = new BigDecimal(has.toString());
Double isuse = b1.subtract(b2).doubleValue();
// BigDecimal isuse1 = new BigDecimal(isuse.toString());
Double res = b2.divide(b1, 3, BigDecimal.ROUND_HALF_UP).doubleValue();
int i = (int) (Double.parseDouble(res + "") * 100);
return i;
}
private String getSubtract(Double all, Double has) {
BigDecimal b1 = new BigDecimal(all.toString());
BigDecimal b2 = new BigDecimal(has.toString());
Double isuse = b1.subtract(b2).doubleValue();
return isuse + "";
}
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
}
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
switch (v.getId()) {
case R.id.gg_loadmore:
Intent intent = new Intent(getActivity(), TouziActivity.class);
startActivity(intent);
break;
case R.id.item_tt:
if (Default.userId == 0) {
Intent intent1 = new Intent(getActivity(), LoginActivity.class);
startActivity(intent1);
} else {
Intent intent1 = new Intent(getActivity(), BidItem.class);
intent1.putExtra("id", id_tt);
intent1.putExtra("type", shabiphp(type_tt));
startActivity(intent1);
}
break;
case R.id.item_yy:
if (Default.userId == 0) {
Intent intent1 = new Intent(getActivity(), LoginActivity.class);
startActivity(intent1);
} else {
Intent intent2 = new Intent(getActivity(), BidItem.class);
intent2.putExtra("id", id_yy);
intent2.putExtra("type", shabiphp(type_yy));
startActivity(intent2);
}
break;
case R.id.autoVerticalScrollTextView:
getActivity().startActivity(new Intent(getActivity(), NewsListActivity.class));
break;
default:
break;
}
}
public int shabiphp(int i) {
int j = 0;
switch (i) {
case 1:
j = 3;
break;
case 2:
j = 4;
break;
case 3:
j = 5;
break;
case 4:
j = 6;
break;
case 5:
j = 7;
break;
default:
break;
}
return j;
}
@Override
public void onPageScrollStateChanged(int arg0) {
// TODO Auto-generated method stub
}
@Override
public void onPageScrolled(int arg0, float arg1, int arg2) {
// TODO Auto-generated method stub
}
@Override
public void onPageSelected(int arg0) {
index = arg0;
setImage(arg0);
}
@Override
public void onDestroy() {
// TODO Auto-generated method stub
super.onDestroy();
isRunning = false;
}
}
<file_sep>/app/src/main/java/com/ys/www/asscg/activity/MainTabActivity.java
package com.ys.www.asscg.activity;
import android.content.Intent;
import android.content.IntentFilter;
import android.net.ConnectivityManager;
import android.os.Bundle;
import android.support.v4.app.Fragment;
import android.support.v4.app.FragmentActivity;
import android.view.Gravity;
import android.view.KeyEvent;
import android.view.LayoutInflater;
import android.view.View;
import android.widget.FrameLayout;
import android.widget.RadioButton;
import android.widget.RadioGroup;
import android.widget.TextView;
import android.widget.Toast;
import com.ys.www.asscg.R;
import com.ys.www.asscg.fragment.FragmentFactory;
import com.ys.www.asscg.receiver.NetWorkStatusBroadcastReceiver;
import com.ys.www.asscg.util.Default;
/**
* Created by Administrator on 2017/6/22.
*/
public class MainTabActivity extends FragmentActivity {
TextView title_view;
RadioGroup rg_tab;
RadioButton tab_one, tab_two, tab_three;
FrameLayout content_frame;
NetWorkStatusBroadcastReceiver netWorkStatusBroadcastReceiver;
/**
* 当前TabBar 选择项
*/
private int currentIndex = 1;
/***
* 当前TabBar 选择项 之前的选择项
*/
private int lastIndex = 1;
private boolean changeToHomeFlag = false;
public static MainTabActivity mainTabActivity;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.layout_main);
mainTabActivity = this;
init();
}
public void init() {
title_view = findViewById(R.id.title_view);
rg_tab = findViewById(R.id.rg_tab);
tab_one = findViewById(R.id.tab_one);
tab_two = findViewById(R.id.tab_two);
tab_three = findViewById(R.id.tab_three);
content_frame = findViewById(R.id.content_frame);
netWorkStatusBroadcastReceiver = new NetWorkStatusBroadcastReceiver();
IntentFilter filter = new IntentFilter();
filter.addAction(ConnectivityManager.CONNECTIVITY_ACTION);
registerReceiver(netWorkStatusBroadcastReceiver, filter);
Fragment fragment = FragmentFactory.getInstanceByIndex(1);
getSupportFragmentManager().beginTransaction().replace(R.id.content_frame, fragment).commit();
title_view.setText("理财");
tab_one.setChecked(true);
rg_tab.setOnCheckedChangeListener(new RadioGroup.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(RadioGroup group, int checkedId) {
/**
* 判断用户是否登陆 , 借款和个人账户的跳转选择
*/
int selectedId = 0;
switch (checkedId) {
case R.id.tab_one:
selectedId = 1;
break;
case R.id.tab_two:
selectedId = 2;
break;
case R.id.tab_three:
selectedId = 3;
break;
default:
break;
}
lastIndex = currentIndex;
currentIndex = selectedId;
changeTitle(selectedId);
Fragment fragment = FragmentFactory.getInstanceByIndex(selectedId);
// if (changeToHomeFlag) {
// HomeFragment homeFragment = (HomeFragment) fragment;
// changeToHomeFlag = false;
// }
getSupportFragmentManager().beginTransaction().replace(R.id.content_frame, fragment)
.commitAllowingStateLoss();
}
});
}
/**
* 更改标题
*
* @param index Tab 选项
*/
private void changeTitle(int index) {
switch (index) {
case 1:
title_view.setText("理财");
break;
case 2:
if (Default.userId == 0) {
Intent userInfoLoginIntent = new Intent();
userInfoLoginIntent.setClass(this, LoginActivity.class);
startActivity(userInfoLoginIntent);
} else {
title_view.setText("个人账户");
}
break;
case 3:
title_view.setText("设置");
break;
default:
break;
}
}
/***
* 跳转到首页
*
* @return
*/
public void IndexView() {
tab_one.setChecked(true);
}
private long lastTime;
private int closeNum;
@Override
protected void onDestroy() {
super.onDestroy();
unregisterReceiver(netWorkStatusBroadcastReceiver);
}
@Override
public boolean onKeyDown(int keyCode, KeyEvent event) {
// TODO Auto-generated method stub
if (keyCode == KeyEvent.KEYCODE_BACK && event.getRepeatCount() == 0) {
if (event.getKeyCode() == KeyEvent.KEYCODE_BACK) {
closeNum++;
if (System.currentTimeMillis() - lastTime > 5000) {
closeNum = 0;
}
lastTime = System.currentTimeMillis();
if (closeNum == 1) {
finish();
} else {
showCustomToast(R.string.exit);
}
}
return false;
}
return super.onKeyDown(keyCode, event);
}
public void showCustomToast(String text) {
View toastRoot = LayoutInflater.from(MainTabActivity.this).inflate(R.layout.common_toast, null);
((TextView) toastRoot.findViewById(R.id.toast_text)).setText(text);
Toast toast = new Toast(MainTabActivity.this);
toast.setGravity(Gravity.CENTER, 0, 0);
toast.setDuration(Toast.LENGTH_SHORT);
toast.setView(toastRoot);
toast.show();
}
public void showCustomToast(int id) {
showCustomToast(getResources().getString(id).toString());
}
}
<file_sep>/app/src/main/java/com/ys/www/asscg/fragment/AccountFragment_Scg.java
package com.ys.www.asscg.fragment;
import android.animation.Animator;
import android.animation.AnimatorSet;
import android.animation.ObjectAnimator;
import android.animation.ValueAnimator;
import android.annotation.SuppressLint;
import android.content.Intent;
import android.os.Bundle;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.view.View.OnClickListener;
import android.view.ViewGroup;
import android.view.animation.DecelerateInterpolator;
import android.view.animation.LinearInterpolator;
import android.widget.ImageView;
import android.widget.TextView;
import com.squareup.picasso.Picasso;
import com.ys.www.asscg.R;
import com.ys.www.asscg.activity.PeopleInfoDataActivity;
import com.ys.www.asscg.base.BaseFragment;
import com.ys.www.asscg.http.HttpClient;
import com.ys.www.asscg.util.Default;
import com.ys.www.asscg.view.ChuckWaveView;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.ArrayList;
import java.util.List;
//苏常账户
@SuppressLint("NewApi")
public class AccountFragment_Scg extends BaseFragment implements OnClickListener {
private ChuckWaveView mWaveView = null;
private float mCurrentHeight = 0;
TextView total_rs, freeze_rs, user_name_rs;
ImageView user_pic;
int jxj_num = 0;
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
View mainView = inflater.inflate(R.layout.layout_account, null);
mainView.findViewById(R.id.item_personalset).setOnClickListener(this);
mainView.findViewById(R.id.item_tzrecord).setOnClickListener(this);
mainView.findViewById(R.id.item_jyjl).setOnClickListener(this);
mainView.findViewById(R.id.cz_rs).setOnClickListener(this);
mainView.findViewById(R.id.tx_rs).setOnClickListener(this);
mainView.findViewById(R.id.item_jxj).setOnClickListener(this);
mainView.findViewById(R.id.item_redpkg).setOnClickListener(this);
total_rs = (TextView) mainView.findViewById(R.id.total_rs);
freeze_rs = (TextView) mainView.findViewById(R.id.freeze_rs);
user_pic = (ImageView) mainView.findViewById(R.id.user_pic);
user_name_rs = (TextView) mainView.findViewById(R.id.user_name_rs);
mWaveView = (ChuckWaveView) mainView.findViewById(R.id.main_wave_v1);
initview();
return mainView;
}
private void initview() {
// TODO Auto-generated method stub
loadWaveData(0.30f);
}
private void loadWaveData(float height) {
float a = 0.33f;
height = a;
// Toast.makeText(MainActivity.this, height + "", 1000).show();
final List<Animator> mAnimators = new ArrayList<Animator>();
ObjectAnimator waveShiftAnim = ObjectAnimator.ofFloat(mWaveView, "waveShiftRatio", 0f, 1f); // 水平方向循环
waveShiftAnim.setRepeatCount(ValueAnimator.INFINITE);
waveShiftAnim.setDuration(2000);
waveShiftAnim.setInterpolator(new LinearInterpolator());
mAnimators.add(waveShiftAnim);
ObjectAnimator waterLevelAnim = ObjectAnimator.ofFloat(mWaveView, "waterLevelRatio", mCurrentHeight, height); // 竖直方向从0%到x%
waterLevelAnim.setDuration(6000);
waterLevelAnim.setInterpolator(new DecelerateInterpolator());
mAnimators.add(waterLevelAnim);
mWaveView.invalidate();
AnimatorSet mAnimatorSet = new AnimatorSet();
mAnimatorSet.playTogether(mAnimators);
mAnimatorSet.start();
mCurrentHeight = height;
}
@Override
public void onResume() {
// TODO Auto-generated method stub
super.onResume();
new Thread() {
@Override
public void run() {
super.run();
doHttpUpdatePeopleInfo();
}
}.start();
}
public void doHttpUpdatePeopleInfo() {
String url = Default.ip + Default.peoInfoUpdate;
try {
String s = HttpClient.post(url, null);
if (!"".equals(s)) {
JSONObject response = str2json(s);
if (response.getInt("status") == 1) {
// updateUserInfo(response);
total_rs.setText(response.getString("total") + "元");
// 冻结
freeze_rs.setText(response.getString("freeze") + "元");
Picasso.with(getActivity()).load(Default.ip + response.getString("head")).into(user_pic);
user_name_rs.setText(response.getString("username"));
} else {
showCustomToast(response.getString("message"));
}
} else {
showCustomToast("获取失败");
}
Log.e("HttpClient", s);
} catch (Exception e) {
e.printStackTrace();
}
}
public JSONObject str2json(String s) {
JSONObject json = null;
try {
json = new JSONObject(s);
} catch (JSONException e) {
e.printStackTrace();
}
return json;
}
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.item_personalset:// 用户设置
Intent intent = new Intent(getActivity(), PeopleInfoDataActivity.class);
startActivity(intent);
break;
case R.id.item_tzrecord:// 投资记录
// Intent intent1 = new Intent(getActivity(), InvestManagerStandardActivity.class);
// startActivity(intent1);
break;
case R.id.item_jyjl:// 交易记录
// startActivity(new Intent(getActivity(), PeopleInfoJiaoYi_New.class));
break;
case R.id.cz_rs:// 充值
// Intent i = new Intent(getActivity(), ChoosePayType.class);
// startActivity(i);
break;
case R.id.tx_rs:// 提现
getUserBankCard();
break;
case R.id.item_jxj:// 加息劵
// Intent i1 = new Intent(getActivity(), InterestratesecuritiesActivity.class);
// startActivity(i1);
break;
case R.id.item_redpkg://红包
// Intent i2 = new Intent(getActivity(), MyBriberyMoney.class);
// startActivity(i2);
break;
default:
break;
}
}
public void getUserBankCard() {
}
;
// 解析获取到银行卡信息
// public void decoidTheUesrCardInfo(JSONObject json) {
// try {
// if (json.getString("bank_num") != null && !json.getString("bank_num").equals("")) {
//
// Intent intent = new Intent(getActivity(), peopleInfoWithdrawalActivity.class);
//
// intent.putExtra("bank_num", json.getString("bank_num"));
//
// if (json.getString("bank_name") != null && !json.getString("bank_name").equals("")) {
//
// intent.putExtra("bank_name", json.getString("bank_name"));
//
// }
// if (json.getString("real_name") != null && !json.getString("real_name").equals("")) {
//
// intent.putExtra("real_name", json.getString("real_name"));
// }
// // if (json.getString("all_money") != null
// // && !json.getString("all_money").equals("")) {
// intent.putExtra("all_money", json.getString("all_money"));
// MyLog.e("提现准备传的钱数", json.getString("all_money"));
// // }
// if (json.getString("qixian") != null && !json.getString("qixian").equals("")) {
//
// intent.putExtra("qixian", json.getString("qixian"));
// }
//
// startActivity(intent);
//
// }
// } catch (JSONException e) {
// // TODO Auto-generated catch block
// e.printStackTrace();
// }
//
// }
}
| 8b57fef91e547e64e0dae0935b94e6b352ed09d5 | [
"Java",
"Gradle"
] | 10 | Gradle | NikemoXss/AsScg | cee33bbafd84a9fffc83d30cbb7f2839d2717358 | bdc80d19d6529d5df555304e21d6152f79242b78 | |
refs/heads/master | <file_sep># 更新日志
## 卡寄售 v1.0.3 - Updated on 2016.5.24
* 调整页面架构
* fulu.js页面性能优化。
## 卡寄售 v1.0.2 - Updated on 2016.5.24
* 新增添加订单动画效果(改成葫芦娃)
* 修复部分手机上,打开服务条款时,js报错。关闭debug模式
* 修复部分手机上售卡成功页面只有frame的显示情况。修复主页面先show出来,然后$nextTick openframe页面。
## 卡寄售 v1.0.1 - Updated on 2016.5.23
* 初始版本
* 修复N多提示信息
* 修复验证上限的问题
* 用户输入的或上传的卡密中含有空格换行是不做处理。
<file_sep>var gulp = require('gulp');
var watch = require('gulp-watch');
var config = require('../config');
gulp.task('watch', ['scss'], function () {
watch([config.scss.src, config.image.src], function () { //监听所有scss
gulp.start('scss');
});
//watch(config.image.src, function () { //监听所有image
// gulp.start('image');
//});
//watch(config.script.src, function () { //监听所有js
// gulp.start('script');
//});
//watch(config.html.src, function () { //监听所有js
// gulp.start('html');
//});
})<file_sep>var baseDpr = 3;
module.exports = {
px2rem: {
baseDpr: baseDpr, // base device pixel ratio (default: 2)
threeVersion: false, // whether to generate @1x, @2x and @3x version (default: false)
remVersion: true, // whether to generate rem version (default: true)
remUnit: 1080, // rem unit value (default: 75)
remPrecision: 6 // rem precision (default: 6)
},
autoprefixer: {},
minifyCss: {
advanced: false,//类型:Boolean 默认:true [是否开启高级优化(合并选择器等)]
compatibility: 'ie7',//保留ie7及以下兼容写法 类型:String 默认:''or'*' [启用兼容模式; 'ie7':IE7兼容模式,'ie8':IE8兼容模式,'*':IE9+兼容模式]
keepBreaks: false//类型:Boolean 默认:false [是否保留换行]
},
base64: {
extensions: ['png', 'ttf'],
maxImageSize: 100000, // bytes
},
htmlOne: {
removeSelector: '', // selector 配置,源文档中符合这个selector的标签将自动移除
keepliveSelector: 'script', // 不要combo的selector配置,源文档中符合这个selector的将保持原状。如果是相对路径,则会根据配置修改成线上可访问地址
destDir: './', // dest 目录配置,一般情况下需要和 gulp.dest 目录保持一致,用于修正相对路径资源
coimport: true, // 是否需要把 @import 的资源合并
cssminify: true, // 是否需要压缩css
jsminify: true, // 是否需要压缩js
publish: false, // 是否是发布到线上的文件,false为发布到日常,预发理论上和日常的文件内容一样
appName: 'app-xxx', //仓库名
appVersion: '0.0.0', //发布版本号
noAlicdn: false, // js相对路径拼接的域名是否是alicdn的
onlinePath: '', //非alicdn线上域名
dailyPath: '', //非alicdn日常域名
}
}<file_sep>var gulp = require('gulp');
var sass = require('gulp-sass');
var px2rem = require('gulp-px3rem');
var rename=require('gulp-rename');
var handleErrors = require('../util/handleErrors');
var autoprefixer = require('gulp-autoprefixer');
var base64 = require('gulp-base64');
var config = require('../config').scss;
var plugin = require('../plugin');
gulp.task('scss', function () {
gulp.src(config.src)
.pipe(sass(config.settings))
.on('error', handleErrors)
.pipe(px2rem(plugin.px2rem))
.pipe(autoprefixer(plugin.autoprefixer))
.pipe(base64(plugin.base64))
.pipe(rename(plugin.base64))
.pipe(gulp.dest(config.dest))
});<file_sep>module.exports = {
now: new Date(),
year: new Date().getFullYear(),
month: (new Date().getMonth() + 1) < 10 ? "0" + (new Date().getMonth() + 1) : (new Date().getMonth() + 1),
day: new Date().getDate() < 10 ? "0" + new Date().getDate() : new Date().getDate(),
week: new Date().getDay() < 10 ? "0" + new Date().getDay() : new Date().getDay(),
hours: new Date().getHours() < 10 ? "0" + new Date().getHours() : new Date().getHours(),
minutes: new Date().getMinutes() < 10 ? "0" + new Date().getMinutes() : new Date().getMinutes(),
seconds: new Date().getSeconds() < 10 ? "0" + new Date().getSeconds() : new Date().getSeconds(),
dateFormat: function () {
return this.year.toString() + "_" + this.month.toString() + this.day.toString() + "_" + this.week + "_" + this.hours.toString() + this.minutes.toString() + this.seconds.toString()
},
dateFormatContinuous: function () {
return this.year.toString() + this.month.toString() + this.day.toString() + this.week + this.hours.toString() + this.minutes.toString() + this.seconds.toString()
}
}<file_sep>var gulp = require('gulp');
var del = require('del');
var root = require('../config').root;
/**
* clear 清除文件夹任务
* */
// 清除dist
gulp.task('clean:dist', function () {
return del([root.dist.root]);
});
// 清除build
gulp.task('clean:build', function () {
return del([root.build]);
});
| 4c4aeb670e2671f4b5e8ac6f6cbc10114accad27 | [
"Markdown",
"JavaScript"
] | 6 | Markdown | AnyGong/FuelCard | 7e8e8bdecbf2dfdb579530f1ebbabd6a53c0aae3 | 41be42d116f159767cad6260d4b5a21353b605ee | |
refs/heads/master | <repo_name>kasper084/discriminant-tests<file_sep>/src/main/java/some/discriminant/util/Discriminant.java
package some.discriminant.util;
public class Discriminant {
public static double calculate(double a, double b, double c) {
double dis = Math.pow(b, 2) - (4 * a * c);
return dis;
}
}<file_sep>/src/main/java/some/discriminant/DiscriminantApplication.java
package some.discriminant;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class DiscriminantApplication {
public static void main(String[] args) {
SpringApplication.run(DiscriminantApplication.class, args);
}
}
| ffc46526500bf837e7c0e41bd430f2e968dde028 | [
"Java"
] | 2 | Java | kasper084/discriminant-tests | a1829146a1980de248fc07b9af2dc874e296e62e | 7047642a50e451bd6c877043f1cefbf6142b0b68 | |
refs/heads/master | <repo_name>manu-msr/java-noob<file_sep>/Tienda/Aplicacion.java
package Proyecto; //Paquete del archivo
import java.util.Scanner;
/**
* PROYECTO FINAL - ICC
* Programa para que maneja una tienda
* @author <NAME> Ciencias UNAM
* @version 1.01 Diciembre 2012
*/
public class Aplicacion{
public static void main(String[] args){
/**
* Clase Tienda, contiene en su constructor
* los archivos .ser y .txt los cuáles se abren
* al crear el objeto.
*/
Tienda tienda = new Tienda();
Scanner teclado = new Scanner(System.in);
/**
* Menú de opciónes para controlar el sistema
*/
int opcion = 1; //Variable que controlará el menú.
do{
System.out.println("\nSelecciona una Opción: "
+"\n[1]Consular existencias de algún artículo."
+"\n[2]Dar de alta un nuevo artículo."
+"\n[3]Registrar una nueva venta."
+"\n[4]Mostrar empleados."
+"\n[5]Dar de alta un nuevo empleado."
+"\n[6]Mostrar las ventas."
+"\n[7]Salir del sistema.");
String op = teclado.nextLine();
opcion = Integer.parseInt(op); //Se hace el cambio para evitar posibles errores con las clases
//y métodos relacionados con la clase Tienda.
switch(opcion){ //Switch para manejar las opciones del menú
//Caso 1: Consular existencias de algún artículo
case 1:
tienda.consultarExistencias();
break;
//Caso 2: Dar de alta un nuevo artículo
case 2:
tienda.darDeAltaArt();
break;
//Caso 3: Registrar una nueva venta
case 3:
tienda.registrarVenta();
break;
//Caso 4: Mostrar empleados
case 4:
tienda.mostrarEmpleados();
break;
//Caso 5: Dar de alta un nuevo empleado
case 5:
tienda.darDeAltaEmp();
break;
//Caso 6: Mostrar las ventas
case 6:
tienda.mostrarVentas();
break;
//Salir del sistema
case 7:
tienda.salir();
break;
//En caso de que ponga una opción incorrecta
default:
System.out.println("Opción incorrecta");
break;
}
} while (opcion != 7); //Mientras la opción no sea 7
}
}<file_sep>/Matrices2/TDAMatriz.java
public interface TDAMatriz{
public int getRenglones();
public int getColumnas();
public void agrega(int i, int j, Double elemento);
public Double get(int i, int j);
public TDAMatriz suma(TDAMatriz otra);
public TDAMatriz multiplicaEscalar(int e);
public TDAMatriz traspuesta();
public boolean equals(Object objeto);
}<file_sep>/Tienda/Pelicula.java
package Proyecto; //Paquete donde se encuentra el proyecto
/**
* Clase Pelicula.
* Hereda de Disco que a su vez hereda de Articulo
* @author <NAME> Ciencias UNAM
* @version 1.04 Diciembre 2012
*/
public class Pelicula extends Disco{
//Atributos
protected String formato;
protected String genero;
protected int anio;
/**
* Constructor por omisión
* @throws TiendaException -- De la clase padre
*/
public Pelicula() throws TiendaException{
super();
formato = "";
genero = "";
anio = 1000;
}
/**
* Constructor a partir de siete parámetros
* Cuatro de la clase padre y tres de la clase hija
* @param c -- Clave única
* @param e -- Número de existencia
* @param p -- Precio
* @param n -- Nombre
* @param f -- Formato (DVD, Blu-Ray)
* @param g -- Genero
* @param a -- Año de salida
* @throws TiendaException -- Verifica que el Formato
* y año de salida se al correcto
*/
public Pelicula(String c, int e, double p, String n, String f, String g, int a) throws TiendaException{
super(c,e,p,n);
if((f.equalsIgnoreCase("DVD") || f.equalsIgnoreCase("BLU-RAY")) && a>1000){
formato = f;
genero = g;
anio = a;
} else {
throw new TiendaException("El formato debe ser DVD o BLUE-RAY y el año mayor a 1000.");
}
}
/**
* Método de acceso
* Obtiene el formato
* @return String -- Formato
*/
public String obtenerFormato(){
return formato;
}
/**
* Método de acceso
* Obtiene el género
* @return String -- Género
*/
public String obtenerGenero(){
return genero;
}
/**
* Método de acceso
* Obtiene el año de salida
* @return int -- Año de salida
*/
public int obtenerAnio(){
return anio;
}
/**
* Método modificador a partir de un parámetro
* Establece el Formato
* @param f -- Formato
* @throws TiendaException -- Revisa que el formato
* sea el correcto
*/
public void establecerFormato(String f) throws TiendaException{
if(f.equalsIgnoreCase("DVD") || f.equalsIgnoreCase("BLU-RAY")) formato = f;
else throw new TiendaException("El formato debe ser DVD o BLUE-RAY. Intenta de nuevo");
}
/**
* Método modificador a partir de un parámetro
* Establece el género
* @param g -- Género
*/
public void establecerGenero(String g){
genero = g;
}
/**
* Método modificador a partir de un parámetro
* Establece el año
* @param a -- Anio
* @throws Tienda Exception -- Verifica que el
* año sea correcto
*/
public void establecerAnio(int a) throws TiendaException{
if(a>1000) anio = a;
else new TiendaException("Demasidado antiguo... Prueba con un año mayor que 1000.");
}
/**
* Método toString
* Imprime la Película
* @return String -- Película
*/
public String toString(){
return super.toString()+"\nFormato: "+formato+"\nGénero: "+genero+"\nAño de salida: "+anio;
}
}<file_sep>/Fracciones/PruebaFracciones.java
/**
* Programa para probar la clase fraccion.
* @author <NAME>.
* @version 1.00 Marzo 2013
*/
public class PruebaFracciones {
/**
* Método main
*/
public static void main(String[] args) {
/**
* Crear dos fracciones
*/
System.out.println("Creando dos fracciones...\n");
Fraccion uno = new Fraccion(3628800,759374);
Fraccion dos = new Fraccion(10,5);
/**
* Mostrar las fracciones
*/
System.out.println("Las fracciones son: "
+ "\n" + uno.muestra() + " y " + dos.muestra());
/**
* Operaciones entre las fracciones
*/
System.out.println("\nSuma de las dos fracciones: " + uno.suma(dos).muestra());
System.out.println("Resta de las dos fracciones: "+ uno.resta(dos).muestra());
System.out.println("Multiplicación de las dos fracciones: "+ uno.multiplica(dos).muestra());
System.out.println("División de las dos fracciones: "+ uno.divide(dos).muestra());
/**
* Multiplicar por un escalar
*/
System.out.println("\nMultiplicamos las fracciones por el entero 2:");
System.out.println(uno.muestra() + ": "+uno.multiplicaEscalar(2).muestra());
System.out.println(dos.muestra() +": "+dos.multiplicaEscalar(2).muestra());
/**
* Saber si las fracciones son propias
*/
System.out.println("\nLas fracciones son propias?: ");
System.out.println(uno.muestra() + ": " + uno.esPropia());
System.out.println(dos.muestra() + ": " + dos.esPropia());
/**
* Obtener el valor real de las fracciones
*/
System.out.println("\nValor real de las fracciones: ");
System.out.println(uno.muestra() + ": " + uno.daValorReal());
System.out.println(dos.muestra() + ": " + dos.daValorReal());
/**
* Simplificamos las fracciones
*/
System.out.println("\nSimplificar las fracciones: ");
System.out.println(uno.muestra() + ": " + uno.simplifica().muestra());
System.out.println(dos.muestra() + ": " + dos.simplifica().muestra());
}
}
<file_sep>/Tienda/VendedorArchivoSecuencial.java
package Proyecto; //Paquete donde se encuentra la clase
//Paquetes para usar serialización
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectOutputStream;
import java.util.InputMismatchException;
import java.util.Scanner;
/**
* Clase para crear un archivo secuencial de Empleados
* @author <NAME> Ciencias UNAM
* @version 1.00 Diciembre 2012
*/
public class VendedorArchivoSecuencial{
//Atributos de la clase
private ObjectOutputStream salida;
private String archivo;
/**
* Constructor a partir del nombre del archivo
* @param n -- Nombre del Archivo
*/
public VendedorArchivoSecuencial(String n){
archivo = n;
}
/**
* Método para abrir el archivo
*/
public void abrirArchivo(){
try{
salida = new ObjectOutputStream(new FileOutputStream(archivo, true));
} catch (IOException io){
System.out.println("Error al abrir el archivo");
}
}
/**
* Método que obtiene los datos del Empleado
* @throws TiendaException -- En caso de que ingrese o
* introduzca un dato incorrecto.
*/
public Vendedor pedirDatos(){
Vendedor vendedor = null; //Vendedor que devolverá el método
Scanner teclado = new Scanner(System.in);
try{
boolean agrego = false;
while(agrego == false){
vendedor = new Vendedor();
System.out.print("RFC: ");
vendedor.establecerRFC(teclado.nextLine());
System.out.print("Nombre completo: ");
vendedor.establecerNombre(teclado.nextLine());
System.out.print("Dirección: ");
vendedor.establecerDireccion(teclado.nextLine());
System.out.print("Teléfono: ");
String telefono = teclado.nextLine();
vendedor.establecerTelefono(Integer.parseInt(telefono));
System.out.print("Sección: ");
vendedor.establecerSeccion(teclado.nextLine());
System.out.print("Sueldo: ");
String sueldo = teclado.nextLine();
vendedor.establecerSueldo(Double.parseDouble(sueldo));
//Obtener la clave del vendedor
String clave = vendedor.obtenerNombre().substring(0,4) + vendedor.obtenerRFC().charAt(0)+
vendedor.obtenerRFC().charAt(2)+vendedor.obtenerRFC().substring(4,6);
clave = clave.toLowerCase();
vendedor.establecerClave(clave);
//Muestra los datos ingresados y pregunta si son correctos
System.out.println("Haz ingresado el siguiente empleado:\n"+vendedor.toString());
System.out.println("Los dados son correctos? [S/N]: ");
String resp = teclado.nextLine();
if(resp.equalsIgnoreCase("S")){
agrego = true;
}
}
}catch (TiendaException e){
System.out.println(e.getMessage());
}catch (NumberFormatException e){
System.out.println("Dato Incorrecto");
} catch (InputMismatchException e){
System.out.println("Dato Incorrecto");
}
return vendedor;
}
/**
* Método para agregar Vendedores al archivo
*/
public void agregarVendedores(){
Vendedor vendedor = null;
Scanner teclado = new Scanner(System.in);
String opcion = "S";
do{
System.out.println("Deseas agregar un empleado? [S/N]");
opcion = teclado.nextLine();
if(!opcion.equalsIgnoreCase("S")&&!opcion.equalsIgnoreCase("N")){
System.out.println("Opción incorrecta");
}else
if(opcion.equalsIgnoreCase("S")){
vendedor = pedirDatos();
try {
if (new File(archivo).exists()){
agregarMasArchivos(vendedor);
}else {
abrirArchivo();
salida.writeObject(vendedor);
System.out.println("\nEmpleado agregado exitosamente");
cerrarArchivo();
}
}catch (IOException ex){
System.out.println("No se pudo escribir en el archivo");
}
}
}while(!opcion.equalsIgnoreCase("N"));
}
/**
* Método que agrega un artículo al archivo, sin escribir un código, para ello
* Éste método, abre el archivo, añade el objeto al final del archivo y finalmente lo cierra.
*/
public void agregarMasArchivos(Object o){
try {
ObjectOutputStreamPropio oos = new ObjectOutputStreamPropio(new FileOutputStream(archivo,true));
oos.writeUnshared(o);
oos.close();
}catch (Exception e){
System.out.println("Imposible agregar el articulo al archivo");
}
}
/**
* Método que cierra el archivo y termina la ejecución
*/
public void cerrarArchivo(){
try{
salida.close();
} catch ( IOException ioe ) {
System.out.println( "Error al cerrar el archivo." );
}
}
}<file_sep>/Tienda/Tienda.java
package Proyecto; //Paquete donde se encuentra la clase
/**
* Clase tienda que manejará los artículos y empleados
* @author <NAME>
* @version 1.04 Diciembre 2012
*/
import java.util.Scanner;
public class Tienda{
//Atributos de la clase
ArticuloArchivoSecuencial articuloAgregar; //Agregar articulos
VendedorArchivoSecuencial vendedorAgregar; //Agregar empleados
LeerArticuloSecuencial articuloLeer; //Leer artículos
LeerEmpleadoSecuencial vendedorLeer; //Leer vendedores
Scanner teclado = new Scanner(System.in); //Leer por teclado
GuardarVentas ventasGuardar; //Guardar las ventas
LeerVentas ventasLeer; //Leer las ventas
/**
* Constructor por omisión
*/
public Tienda(){
articuloAgregar = new ArticuloArchivoSecuencial("Articulos.ser");
vendedorAgregar = new VendedorArchivoSecuencial("Empleados.ser");
articuloLeer = new LeerArticuloSecuencial("Articulos.ser");
vendedorLeer = new LeerEmpleadoSecuencial("Empleados.ser");
ventasGuardar = new GuardarVentas("Ventas.txt");
ventasLeer = new LeerVentas("Ventas.txt");
}
/**
* Método para para consultar
* las existencias de algún artículo
*/
public void consultarExistencias(){
int opcion = 1; //Opción que controlará el menú
do{
System.out.println("\nSeleccione un tipo de búsqueda:" +
"\n[1]Por Clave"+
"\n[2]Por Tipo de Artículo"+
"\n[3]Por Nombre"+
"\n[4]Salir"+
"\nOpción: ");
String op = teclado.nextLine();
opcion = Integer.parseInt(op);
switch(opcion){
case 1:
System.out.println("Introduzca la clave del producto");
articuloLeer.abrirArchivo();
articuloLeer.buscarPorClave(teclado.nextLine());
articuloLeer.cerrarArchivo();
break;
case 2:
System.out.println("Seleccione el tipo del artículo: "+
"\n[1]Libro"+
"\n[2]Disco");
String tip = teclado.nextLine();
int tipo = Integer.parseInt(tip);
switch(tipo){
case 1:
articuloLeer.abrirArchivo();
articuloLeer.buscarPorTipo("Libro");
articuloLeer.cerrarArchivo();
break;
case 2:
articuloLeer.abrirArchivo();
articuloLeer.buscarPorTipo("Disco");
articuloLeer.cerrarArchivo();
break;
default:
System.out.println("Opción incorrecta");
}
break;
case 3:
System.out.println("Introducza el nombre del producto:");
articuloLeer.abrirArchivo();
articuloLeer.buscarPorNombre(teclado.nextLine());
articuloLeer.cerrarArchivo();
break;
case 4:
System.out.println("Saliendo de busquedas...");
break;
default:
System.out.println("Opción incorrecta");
break;
}
} while(opcion != 4);
}
/**
* Método para dar de alta un nuevo
* artículo
*/
public void darDeAltaArt(){
articuloAgregar.agregarArticulos();
}
/**
* Método para registrar una nueva venta
*/
public void registrarVenta(){
ventasGuardar.abrirArchivo();
ventasGuardar.agregarVentas();
ventasGuardar.cerrarArchivo();
}
/**
* Método para mostrar todas las ventas
*/
public void mostrarVentas(){
System.out.println("Selecciona una opción:"
+"\n[1]Todas las ventas."
+"\n[2]Por fecha.");
String op = teclado.nextLine();
int opcion = Integer.parseInt(op);
switch(opcion){
//Mostrar todas las ventas
case 1:
ventasLeer.abrirArchivo();
ventasLeer.leerVentas();
ventasLeer.cerrarArchivo();
break;
//Mostrar las ventas de una fecha específica
case 2:
System.out.println("Fecha a buscar: ");
String f = teclado.nextLine();
ventasLeer.abrirArchivo();
ventasLeer.leerVentasFecha(f);
ventasLeer.cerrarArchivo();
break;
default:
System.out.println("Opción no válida");
}
}
/**
* Método que muestra los empleados de la tienda
*/
public void mostrarEmpleados(){
vendedorLeer.abrirArchivo();
vendedorLeer.leerArchivos();
vendedorLeer.cerrarArchivo();
}
/**
* Método para dar de alta un nuevo empleado
*/
public void darDeAltaEmp(){
vendedorAgregar.agregarVendedores();
}
/**
* Método para salir del sistema
*/
public void salir(){
System.out.println("Escriba la fecha");
String fecha = teclado.nextLine();
ventasLeer.abrirArchivo();
ventasLeer.leerVentasDia(fecha);
ventasLeer.cerrarArchivo();
}
}<file_sep>/Matrices2/PruebaMatriz.java
import java.io.FileInputStream;
import java.io.InputStream;
import java.io.IOException;
import javax.xml.parsers.SAXParserFactory;
import javax.xml.parsers.SAXParser;
import javax.xml.parsers.ParserConfigurationException;
import org.xml.sax.helpers.DefaultHandler;
import org.xml.sax.Attributes;
import org.xml.sax.SAXException;
import java.util.Random;
import java.util.Scanner;
/**
* Parser para crear matrices
*/
public class PruebaMatriz extends DefaultHandler {
/**
* Para saber si encontró la etiqueta dimensiona.
*/
boolean dimensiona;
/**
* Para saber si encontró la etiqueta dimensionb.
*/
boolean dimensionb;
/**
* Para saber si encontró la etiqueta elemento.
*/
boolean elemento;
/**
* Guarda el parámetro de la etiqueta dimensiona.
*/
int n;
/**
* Guarda el parámetro de la etiqueta dimensionb.
*/
int m;
/**
* Guarda los elementos como cadena.
*/
String elementos;
/**
* Busca las etiquetas.
*/
public void startElement(String uri, String localName, String qName, Attributes attributes) throws SAXException {
if (qName.equalsIgnoreCase("dimensiona")) {
dimensiona = true;
}
if (qName.equalsIgnoreCase("dimensionb")) {
dimensionb = true;
}
if (qName.equalsIgnoreCase("elementos")) {
elemento = true;
}
}
/**
* Obtiene los valores de las etiquetas.
*/
public void characters(char[] ch, int start, int length) throws SAXException {
if (dimensiona) {
n = Integer.parseInt(new String(ch,start,length).trim());
dimensiona = false;
}
if (dimensionb) {
m = Integer.parseInt(new String(ch,start,length).trim());
dimensionb = false;
}
if (elemento) {
elementos = new String(ch,start,length).trim();
elemento = false;
}
}
/**
* Construye la matriz.
*/
public TDAMatriz getMatriz() {
Double[] arreglo = new Double[elementos.length()];
elementos = elementos.replace(" ", "");
for (int i = 0; i < elementos.length(); i++) {
arreglo[i] = new Double(new String(elementos.charAt(i)+""));
}
int contador = 0;
TDAMatriz nueva = new Matriz(n, m);
for(int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
nueva.agrega(i,j, arreglo[contador++]);
}
}
return nueva;
}
public void parseDocument(InputStream is) {
SAXParserFactory spf = SAXParserFactory.newInstance();
try {
SAXParser sp = spf.newSAXParser();
sp.parse(is, this);
}catch(SAXException se) {
se.printStackTrace();
}catch(ParserConfigurationException pce) {
pce.printStackTrace();
}catch (IOException ie) {
ie.printStackTrace();
}
}
/**
* Método main.
*/
public static void main(String args[]) throws IOException {
/* En caso de que no se le pase nada por consola */
if(args.length == 0){
System.out.println("Debes pasar algún argumento");
System.exit(1);
}
/* Matriz generada a partir del parser */
FileInputStream fis=new FileInputStream("matriz.xml");
PruebaMatriz ep= new PruebaMatriz();
ep.parseDocument(fis);
TDAMatriz a = ep.getMatriz();
/* Matriz generada aleatoriamente */
Random alea = new Random();
TDAMatriz b = new Matriz(a.getRenglones(),a.getColumnas());
for (int i = 0; i < b.getRenglones(); i++) {
for (int j = 0; j < b.getColumnas(); j++) {
b.agrega(i,j, new Double(alea.nextInt(100)));
}
}
/* Mostramos las dos matrices */
System.out.println("Matriz a");
System.out.println(a);
System.out.println("Matriz b");
System.out.println(b);
Scanner teclado = new Scanner(System.in);
/* Respuesta dependiendo del parámetro */
switch(args[0]) {
case "-r":
System.out.println("Renglones: " + a.getRenglones());
break;
case "-c":
System.out.println("Columnas: " + a.getColumnas());
break;
case "-e":
System.out.print("Introduce la posición i: ");
int i = teclado.nextInt();
System.out.print("Introduce la posición j: ");
int j = teclado.nextInt();
System.out.println(a.get(i,j));
break;
case "-mo":
System.out.print("Introduce la posición i: ");
i = teclado.nextInt();
System.out.print("Introduce la posición j: ");
j = teclado.nextInt();
System.out.print("Introduce el nuevo elemento: ");
double e = teclado.nextDouble();
a.agrega(i,j, e);
System.out.println(a);
break;
case "-s":
System.out.println("a + b: ");
System.out.println(a.suma(b));
break;
case "-me":
System.out.print("Introduce el escalar: ");
int es = teclado.nextInt();
System.out.println(a.multiplicaEscalar(es));
break;
case "-t":
System.out.println(a.traspuesta());
break;
case "-i":
if (a.equals(b))
System.out.println("Son iguales");
else
System.out.println("Son distintas");
break;
}
}
} <file_sep>/Pokedex/Pokedex.java
package pokedex;
/**
* Interfaz para manejar un Pokedex y mostrar los datos de algunos Pokémons.
* @author <NAME>
* @version 1.00
*/
public class Pokedex {
/**
* Método a partir de un parámetro que devuelve el nombre de un pokemon a
* partir del número que se le pasa como parámetro.
* @param n -- Número del pokémon.
* @return Nombre del pokémon.
*/
public String obtenerPokemon(int n){
switch(n) {
case 1:
return "Bulbasaur";
case 2:
return "Ivysaur";
case 3:
return "Venusaur";
case 4:
return "Charmander";
case 5:
return "Charmeleon";
case 6:
return "Carizard";
default:
return "Pokemon no encontrado";
}
}
/**
* Método para obtener información sobre si el pokémon puede evolucionar
* @param p -- Nombre del pokémon.
* @return información sobre este
*/
public String obtenerEvolución(String n){
if(n.equalsIgnoreCase("Bulbasaur")) {
return "Ivysaur";
}
if(n.equalsIgnoreCase("Ivysaur")) {
return "Venusaur";
}
if(n.equalsIgnoreCase("Venusaur")) {
return "That creature has no further evolution.";
}
if(n.equalsIgnoreCase("Charmander")) {
return "Charmelon";
}
if(n.equalsIgnoreCase("Charmeleon")) {
return "Charizard";
}
if(n.equalsIgnoreCase("Charizar")) {
return "That creature has no further evolution.";
}
return "That creature is not found in our database.";
}
}
<file_sep>/RegistroPalabras/Nodo.java
/**
* Clase para implementar la representacion de un Nodo como un elemento
* de una Lista Ligada.
* @author <NAME>
* @version Junio 2013
*/
public class Nodo {
protected Object elemento; // elemento a guardar
protected Nodo siguiente; // nodo que guardará el siguiente elemento
/**
* Constructor que recibe como parámetro el valor que contendr� el nuevo Nodo.
* Siguiente se inicializa como null.
* @param valor -- Elemento que tendrá el nuevo Nodo.
*/
public Nodo(Object valor) {
this(valor,null);
}
/**
* Constructor que recibe como parámetros el valor del nuevo Nodo y el siguiente
* Nodo en la Lista que suceder+a al nuevo Nodo.
* @param valor -- Elemento del nuevo Nodo.
* @param n -- Siguiente Nodo del nuevo Nodo
*/
public Nodo(Object valor, Nodo n) {
elemento = valor;
siguiente = n;
}
}<file_sep>/Empleados/Programador.java
package Empleados;
/**
* Clase para manejar programadores,
* heredados de la clase Empleado.
* @author <NAME> ciencias UNAM
* @version 1.0 Noviembre 2012
*/
public class Programador extends Empleado{
//Atributos
protected int lineasDeCodigoPorHora;
protected int lenguajesDomina;
/**
* Constructor por omisión
*/
public Programador(){
lineasDeCodigoPorHora = 0;
lenguajesDomina = 0;
}
/**
* Construtor a partir de seis parámetros
* @param nombre -- Nombre del programador,
* hereda de la clase padre.
* @param cedula -- Cédula del prgoramador,
* hereda de la clase padre.
* @param edad -- Edad del programador.
* hereda de la clase padre.
* @param salario -- Salario del programador,
* hereda de la clase padre.
* @param lineasDeCodigoPorHora -- Líneas de código que
* escribe por hora el programador
* @param lenguajesDomina -- Númerode lenguajes que domina.
*/
public Programador(String nombre, String cedula, int edad, double salario, int lineasDeCodigoPorHora, int lenguajesDomina){
super(nombre, cedula, edad, salario);
this.lineasDeCodigoPorHora = lineasDeCodigoPorHora;
this.lenguajesDomina = lenguajesDomina;
}
/**
* Método para obtener el tipo de programador
* @return String -- Tipo en formato cadena
*/
public String obtenerTipo(){
String tipo = "";
if(lenguajesDomina > 3) tipo = "intermedio";
if(lenguajesDomina > 5) tipo = "avanzado";
return super.obtenerTipo() + " programador nivel "+ tipo;
}
/**
* Método que regresa los datos del prgramador
* en formato cadena.
* @return String -- Datos del programador.
*/
public String toString(){
return super.toString() + "\nLineas de código por hora: "+lineasDeCodigoPorHora+"\nLenguajes que domina: "+lenguajesDomina;
}
}<file_sep>/DiferenciaFechas/Fecha.java
import java.util.Scanner;
/**
* Esta clase calcula la diferencia entre los años ingresados, tomará en
* cuenta los días bisiestos añadiéndolos al cálculo de su diferencia de
* dichos años convertidos en días: diferencia de años + dias bisiestos
*/
public class Fecha {
public int difAnios(int a1, int a2) {
int anioDif, cta, i, anioDias;
anioDif = a2 - a1; // para sacar la diferencia de años
/* Para contar los años bisiestos en un intervalo de fechas */
cta = 0; // llevará la cuenta de años bisiestos
/* Recorrerá del a1 al a2 comprobando si existen años bisiestos los años
bisiestos son divisibles por 4 y 400, ecluyendo los que sean divisibles
por 10 */
for (i = a1; i <= a2; i++) {
if ((i % 4 == 0) && (i % 100 != 0 || i % 400 == 0)) {
cta += 1;
}
}
/* La diferencia de años multiplicada por 365 más la cuenta de número de
años bisiestos nos dará el número de días que habrá entre los años
evaluados */
anioDias = (anioDif * 365) + cta;
return anioDias;
}
/**
* Al primer año se le quitarán los días del intervalo del primer día del
* año a la primera fecha ingresada.
*/
public int diasAnioUno(int d1, int me1, int a1) {
int m1, restoDias1;
m1 = 0;
/* Cada número de mes tiene asignado números pre-calculados ya que no
todos los meses tienen el mismo número de días.
Se empieza con el día 1 y aumenta de acuerdo al número de días que
tiene cada mes hasta completar el año */
switch(me1) {
case 1:
m1 = 0;
break;
case 2:
m1 = 31;
break;
case 3:
m1 = 59;
break;
case 4:
m1 = 90;
break;
case 5:
m1 = 120;
break;
case 6:
m1 = 151;
break;
case 7:
m1 = 181;
break;
case 8:
m1 = 212;
break;
case 9:
m1 = 243;
break;
case 10:
m1 = 273;
break;
case 11:
m1 = 304;
break;
case 12:
m1 = 334;
break;
}
/* Dependiendo del mes asignado sus días se sumará con los días ingresados
de la primera fecha */
restoDias1 = m1 + d1;
return restoDias1;
}
/* Al segundo año se le quitarán los días del intervalo de la segunda fecha
ingresada hasta el último día de ese mismo año. */
public int diasAnioDos(int d2, int me2, int a2) {
int m2, restoDias2;
m2 = 0;
/* Cada número de mes tiene asignado números precalculados ya que no todos
los meses tienen el mismo número de días.
Se empieza con el día 1 y aumenta de acuerdo al número de días que
tiene cada mes hasta completar el año. */
switch (me2){
case 1:
m2 = 0;
break;
case 2:
m2 = 31;
break;
case 3:
m2 = 59;
break;
case 4:
m2 = 90;
break;
case 5:
m2 = 120;
break;
case 6:
m2 = 151;
break;
case 7:
m2 = 181;
break;
case 8:
m2 = 212;
break;
case 9:
m2 = 243;
break;
case 10:
m2 = 273;
break;
case 11:
m2=304;
break;
case 12:
m2=334;
break;
}
restoDias2 = m2 + d2;
/* Devuelve el valor que se pretendía calcular */
return restoDias2;
}
public static void main(String[] args) {
Fecha f = new Fecha();
Scanner in = new Scanner(System.in);
/* Se pedira en esta funcion los datos solicitados por el programa */
int dia1, dia2, mes1, mes2, anio1, anio2, restodias1, restodias2, aniodias, diastotales;
/* Anuncia lo que pedira para la primera fecha*/
System.out.println ("Ingresara datos de la primera fecha:");
System.out.println ("Ingrese el dia"); /* Solicita que ingrese el primer dia */
dia1 = in.nextInt();
System.out.println("Ingrese el mes"); /* Solicita que ingrese el primer mes */
mes1 = in.nextInt();
System.out.println ("Ingrese el anio"); /* Solicita que ingrese el primer anio */
anio1 = in.nextInt();
/* Anuncia lo que pedira para la primera fecha*/
System.out.println ("Ingresara datos de la segunda fecha:");
System.out.println ("Ingrese el dia"); /* Solicita que ingrese el segundo dia */
dia2 = in.nextInt();
System.out.println ("Ingrese el mes"); /* Solicita que ingrese el segundo mes */
mes2 = in.nextInt();
System.out.println ("Ingrese el anio"); /* Solicita que ingrese el segundo anio */
anio2 = in.nextInt();
/*Llamada a la funcion difanios*/
aniodias= f.difAnios(anio1, anio2);
/* Lammada a la funcion diasaniouno*/
restodias1=f.diasAnioUno (dia1, mes1, anio1);
/* Llamada a la funcion diasaniodos*/
restodias2=f.diasAnioDos (dia2, mes2, anio2);
/* Calcula 'diastotales' con los valores que devuelve cada funcion */
diastotales= aniodias + (restodias2 - restodias1);
System.out.println ("\n");
System.out.println(diastotales);
}
} <file_sep>/Canica/PruebaCanica.java
package canica;
import java.awt.Color;
/**
* Programa para probar los métodos de la clase canica
* @author <NAME>
* @version 1.00 Marzo, 2013
*/
public class PruebaCanica {
public static void main(String[] args){
//Crear dos canicas
Canica uno = new Canica();
Canica dos = new Canica();
//Mostrar las canicas
System.out.println("Las canicas son: \n" + uno.toString() + "\n"
+ dos.toString());
//Mostrar si son iguales
System.out.println("\nSon iguales?: " + uno.equals(dos));
//Cambiar el color y número de pasos de las canicas
uno.setColor(Color.yellow);
uno.setPasos(10);
dos.setColor(Color.black);
dos.setPasos(5);
//Subir la canica amarilla 2 pasos.
System.out.println("Subió?: " + uno.avanzar("Arriba", 5));
//Mostrar posición de la canica amarilla ahora
System.out.println("Ahora se encuentra en: " + uno.getPos());
//Bajar la canica amarilla 4 pasos.
System.out.println("Bajó?: " + uno.avanzar("Abajo", 4));
//Mostrar la posición de la canica amarilla ahora
System.out.println("Ahora se encuentra en: " + uno.getPos());
//Mover a la derecha la canica negra 2 pasos.
System.out.println("Se movió?: " + dos.avanzar("Derecha", 2));
//Mostrar posición de la canica negra ahora
System.out.println("Ahora se encuentra en: " + dos.getPos());
//Mover a la izquierda la canica negra 2 pasos.
System.out.println("Se movió?: " + dos.avanzar("Izquierda", 2));
//Mostrar la posición de la canica amarilla ahora
System.out.println("Ahora se encuentra en: " + dos.getPos());
//Mostrar el número de canicas
System.out.println("\nNúmero de canicas: " + Canica.cuantasCanicas());
}
}
<file_sep>/Tienda/Disco.java
package Proyecto; //Paquete donde se encuentra la clase
/**
* Clase disco que hereda de Artículo
* @author <NAME> Ciencias UNAM
* @version 1.03 Diciembre 2012
*/
public class Disco extends Articulo{
//Atributos de la clase
protected String nombre;
/**
* Constructor por omisión
* @throws TiendaException -- De la clase padre
*/
public Disco() throws TiendaException{
super();
nombre = "";
}
/**
* Constructor a partir de cuatro parámetros
* tres de la clase padre y uno de la clase hija
* @param c -- Clase
* @patam e -- Existencias
* @param n -- Nombre del disco
* @trhows TiendaException -- De la clase padre
*/
public Disco(String c, int e, double p, String n) throws TiendaException{
super(c,e,p);
nombre = n;
}
/**
* Método de acceso para obtener el nombre
* @return nombre
*/
public String obtenerNombre(){
return nombre;
}
/**
* Método modificador para establecer el nombre
* @param n -- Nombre
*/
public void establecerNombre(String n){
nombre = n;
}
/**
* Método toString
* @return String -- Disco en formato cadena
*/
public String toString(){
return "\nNombre: "+nombre+super.toString();
}
}<file_sep>/Empleados/PruebaEmpleado.java
package Empleados;
import java.util.Scanner;
/**
* Programa para probar la clase empleado
* y la clase programa de herencia.
* @author <NAME> ciencias UNAM
* @version 1.01 Noviembre 2012
*/
public class PruebaEmpleado{
//Método main
public static void main(String[] args){
/*Pruebas de la clase Empleado*/
//Crear un objeto Empleado sin errores
Empleado emp1 = new Empleado("<NAME>", "manumsr93", 19, 5000.00);
System.out.println(emp1.toString());
System.out.println();
//Crear un objeto Empleado donde el rango de edad no es válido
Empleado emp2 = new Empleado("<NAME>", "manumsr93", 15, 5000.00);
//Crear un objeto Empleado donde el rando del salario no es válido
Empleado emp3 = new Empleado("<NAME>", "manumsr93", 19, 200.00);
/*Pruebas de la clase Programador*/
//Crear un objeto Programador con nivel intermedio
Programador prog1 = new Programador("<NAME>","manumsr93", 19, 5000.00, 1000, 5);
System.out.println(prog1.toString());
System.out.println();
//Crear un objeto Programador con nivel avanzado
Programador prog2 = new Programador("<NAME>","manumsr93",19, 15000.00, 1000, 10);
System.out.println(prog2.toString());
}
}
<file_sep>/Robot/Robot.java
package robot;
/**
* Clase para manejar los saludos de un robot.
* @autor <NAME> Ciencias UNAM
* @version 1.00 Febrero 2013
*/
public class Robot {
/**
* Variable de clase <code>name</code> que guardará el
* nombre del robot.
*/
private String name;
/**
* Variable de clase <code>greeting</code> que guardará lo que
* dirá el robot al saluda.
*/
private String greeting;
/**
* Constructor a partir de un parámetro.
* @param nom -- Nombre del robot.
*/
public Robot(String nom) {
name = nom;
greeting = "Konnichiwa";
}
/**
* Constructor a partir de dos parámetros.
* @param nom -- Nombre del robot.
* @param sal -- Saludo.
*/
public Robot(String nom, String sal) {
name = nom;
greeting = sal;
}
/**
* Método que regresa una cadena.
* @return String -- greeting.
*/
public String greet() {
return greeting;
}
/**
* Método a partir de un parámetro que regresa
* una cadena.
* @param cad -- Cadena que recibe como parámetro.
* @return String -- resultado de la concatenación.
*/
public String greetPerson(String cad) {
return greeting + " " + cad;
}
/**
* Método que regresa la concatenación de las variables de clase
* @return String -- Concatenación de las variables.
*/
public String fullGreeting() {
return greeting + ", my name is, " + name
+"\n\t Nice to meet you";
}
}<file_sep>/Tienda/LeerEmpleadoSecuencial.java
package Proyecto; //Paquete donde se encuentra la clase
//Paquetes para utilizar serialización
import java.io.EOFException;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.ObjectInputStream;
/**
* Clase para leer los Empleados de un archivo
* @author <NAME>
* @version 1.01 Diciembre 2012
*/
public class LeerEmpleadoSecuencial{
//Atributos de la clase
private ObjectInputStream entrada;
private String nombre;
/**
* Constructor a partir de un parámetro
* @param archivo -- Nombre del archivo.
*/
public LeerEmpleadoSecuencial(String archivo){
nombre = archivo;
}
/**
* Método para abrir el archivo
*/
public void abrirArchivo(){
try{
entrada = new ObjectInputStream(new FileInputStream( nombre ));
} catch (IOException ioe){
System.out.println("Error al abrir el archivo.");
}
}
/**
* Método para leer los archivos
*/
public void leerArchivos(){
Vendedor vendedor;
try {
while (true){
Object objeto = entrada.readObject();
if(objeto instanceof Vendedor){
vendedor = (Vendedor)objeto;
System.out.println(vendedor.toString());
}else{
System.out.println("No es un artículo");
}
}
}catch (EOFException eofe){
return;
}catch (ClassNotFoundException cnfe){
System.out.println("No se pudo crear el objeto.");
} catch ( IOException ioException ){
System.out.println("Error al leer del archivo.");
System.out.println("La causa " + ioException.toString());
}
}
/**
* Método para cerrar el archivo
*/
public void cerrarArchivo(){
try{
entrada.close();
} catch (IOException ioException){
System.out.println( "Error al cerrar el archivo." );
}
}
}<file_sep>/Fracciones/ServFracciones.java
/**
* Presenta los servicios que ofrece una clase que represente a fracciones.
* @author <NAME>.
* @version 0.1.
* @date Feb 20,2013.
*/
public interface ServFracciones {
/**
* Le suma una fracción a la fracción this.
* @param la fracción a sumar.
* @return la suma de las fracciones.
*/
public Fraccion suma(Fraccion otra);
/**
* Le resta una fracción a la fracción this.
* @param la fracción a sumar.
* @return la resta de las fracciones.
*/
public Fraccion resta(Fraccion otra);
/**
* Multiplica una fraccion por la fraccion this.
* @param la fraccion a multiplicar.
* @return la multiplicación de las fracciones.
*/
public Fraccion multiplica(Fraccion otra);
/**
* Multiplica un entero por la fracción this.
* @param el entero a multiplicar.
* @return el resultado de la multiplicación, que es una fracción.
*/
public Fraccion multiplicaEscalar(int valor);
/**
* Divide la fracción this entre la fracción dada.
* @param la fraccion entre la cual dividir..
* @return El resultado de la division de las fracciones.
*/
public Fraccion divide(Fraccion otra);
/**
* Responde si la fraccion this es propia o no.
* @return true si la fraccion es propia.
*/
public boolean esPropia();
/**
* Lleva a cabo la división del numerador entre el denominador de
* la fracción this.
* @return el real que es resultado de la división
*/
public double daValorReal();
/**
* Simplifica la fracción this.
* @return la fracción simplificada.
*/
public Fraccion simplifica();
/**
* Construye una cadena con una representación adecuada de la fracción this.
* @return la fracción simplificada
*/
public String muestra();
}
<file_sep>/Baraja/Carta.java
/**
* Carta.java
* La clase Carta representa una carta de juego.
* @author <NAME>
* @version 1.00 Octubre 2014
*/
public class Carta {
/**
* Cara de la carta ("As", "Dos",...).
*/
private String cara;
/**
* Palo de la carta ("Corazones", "Diamantes", ...).
*/
private String palo;
/**
* Constructor a partir de dos parámetros.
* @param cara Cara de la carta
* @param palo Palo de la carta
*/
public Carta(String cara, String palo) {
this.cara = cara;
this.palo = palo;
}
/**
* Representación de una carta como cadena.
* @return String - Carta en formato cadena
*/
public String toString() {
return cara + " de " + palo;
}
}<file_sep>/Robot/PruebaRobot.java
package robot;
/**
* Programa que maneja dos robots.
* @author <NAME> Ciencias UNAM
* @version 1.00 Febrero 2013.
*/
public class PruebaRobot {
/**
* Método main
*/
public static void main(String[] args) {
Robot uno = new Robot("GLaDOS", "Welcome to Apperture Science");
Robot dos = new Robot("Wheatly", "Hola amigo!, abre la puerta!");
/**
* Probar los métodos
*/
System.out.println(uno.greet());
System.out.println(uno.greetPerson("Manuel"));
System.out.println(uno.fullGreeting());
System.out.println(dos.greet());
System.out.println(dos.greetPerson("Manuel"));
System.out.println(dos.fullGreeting());
}
}<file_sep>/GUI/LabelFrame.java
import java.awt.FlowLayout; // especifica como se van a ordenar los componentes
import javax.swing.JFrame; // proporciona las características básicas de una ventana
import javax.swing.JLabel; // muestra texto e imágenes
import javax.swing.SwingConstants; // constantes comunes utilizadas con Swing.
import javax.swing.Icon; // interfaz utilizada para manipular imágenes
import javax.swing.ImageIcon; // carga las imágenes
/**
* Programa que muestra texto e imágenes en una ventana.
* Objetivo: Introducir el concepto de GUI.
* @author <NAME>
* @version 1.00
*/
public class LabelFrame extends JFrame {
private JLabel etiqueta1; // JLabel sólo con texto;
private JLabel etiqueta2; // JLabel construida con texto y un icono
private JLabel etiqueta3; // Jlabel con texto adicional e icono
/**
* El constructor de LabelFrame agrega objetos JLabel a JFrame
*/
public LabelFrame() {
super("Prueba de JLabel");
setLayout(new FlowLayout()); // establece el esquema del marco
// Constructor de JLabel con un argumento String
etiqueta1 = new JLabel("Etiqueta con texto");
etiqueta1.setToolTipText("Esta es etiqueta1");
add(etiqueta1);
/* Constructor de JLabel con argumentos de cadena, Icono y
alineación */
Icon unam = new ImageIcon(getClass().getResource("unam.jpg"));
etiqueta2 = new JLabel("Etiqueta con texto e icono", unam,
SwingConstants.LEFT);
etiqueta2.setToolTipText("Esta es etiqueta2");
add(etiqueta2);
etiqueta3 = new JLabel(); // Constructor de JLabel sin argumentos
etiqueta3.setText("Etiqueta con icono y texto en la parte inferior");
etiqueta3.setIcon(unam);
etiqueta3.setHorizontalTextPosition(SwingConstants.CENTER);
etiqueta3.setVerticalTextPosition(SwingConstants.BOTTOM);
etiqueta3.setToolTipText("Esta es etiqueta3");
add(etiqueta3);
}
public static void main(String[] args) {
LabelFrame marcoEtiqueta = new LabelFrame();
marcoEtiqueta.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
marcoEtiqueta.setSize(275, 180);
marcoEtiqueta.setVisible(true);
}
}<file_sep>/Tienda/Juego.java
package Proyecto; //Paquete donde se encuentra la clase
/**
* Clase Juegos
* Hereda de disco y esta a su vez hereda de Artículo
* @author <NAME> Ciencias UNAM
* @version 1.02 Diciembre 2012
*/
public class Juego extends Disco{
//Atributos
protected String tipo;
protected String consola;
/**
* Constructor por omisión
* @throws Tienda Exception -- De la clase padre
*/
public Juego() throws TiendaException{
super();
tipo = "";
consola = "";
}
/**
* Constructor a partir de seis parámetros.
* Tres de la clase padre y tres de la clase hija.
* @param c -- Clave.
* @param e -- Número de Existencia.
* @param p -- Precio.
* @param n -- Nombre.
* @param t -- Tipo.
* @param j -- Consola.
* @param TiendaException -- Exception de la clase padre.
*/
public Juego(String c, int e, double p, String n, String t, String j) throws TiendaException{
super(c,e,p,n);
tipo = t;
consola = j;
}
/**
* Método de acceso
* Obtiene el tipo de juego
* @return String -- Tipo de juego
*/
public String obtenerTipo(){
return tipo;
}
/**
* Método de acceso
* Obtiene la consola
* @return String -- Consola
*/
public String obtenerConsola(){
return consola;
}
/**
* Método modificador a partir de un parámetro
* Establece el tipo de juego
* @param t -- Tipo de juego
*/
public void establecerTipo(String t){
tipo = t;
}
/**
* Método modificador a partir de un parámetro
* Establece la consola
* @param c -- Consola
*/
public void establecerConsola(String c){
consola = c;
}
/**
* Método toString
* Imprime el videojuego
* @return String -- Juego
*/
public String toString(){
return super.toString()+"\nTipo: "+tipo+"\nConsola: "+consola;
}
}<file_sep>/RegistroPalabras/RegistroPalabras.java
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
import java.util.Iterator;
/**
* Clase que cuenta el número de palabras con un tamaño específico de un
* texto.
* @author <NAME>
* @version Junio 2013
*/
public class RegistroPalabras {
/**
* Lista para guardar las palabras del archivo.
*/
private Lista palabras;
/**
* Matriz que guardará los datos.
*/
private int[][] tabla;
/**
* Variable que guarda el tamaño de la palabra de mayor tamaño.
*/
private int mayor;
/**
* Constructor por omisión.
*/
public RegistroPalabras() {
palabras = new Lista();
mayor = 0;
}
/**
* Método que lee el archivo y guarda las palabras en la lista.
* @param archivo -- Nombre del archivo a leer
*/
public void leerArchivo(File lectura) throws IOException {
BufferedReader entrada = new BufferedReader(new FileReader(lectura));
String renglon;
String cadena = ""; // cadena que guardará las palabras
while ((renglon = entrada.readLine()) != null) {
for (int i = 0; i < renglon.length(); i++) {
cadena += renglon.charAt(i); // arma la cadena
System.out.print(renglon.charAt(i));
if(renglon.charAt(i) == ' ') {
agregarLista(cadena); //agrega la cadena a la lista
cadena = ""; // vacía la cadena para armar otra palabra
}
}
agregarLista(cadena);
System.out.println("");
}
}
/**
* Agregar los datos de la lista en la tabla.
*/
public void crearTabla() {
int contador = 0;
tabla = new int[2][mayor]; // crea una tabla de 2 x el tamaño mayor
for(int i = 0; i < mayor; i++) { // llena primera fila
tabla[0][i] = (i+1);
}
for(int i = 0; i < mayor; i++) { // llena segunda fila
tabla[1][i] = (palabras.cuenta(i+1));
}
}
/**
* Método que recorre la tabla y muestra los datos almacenados en ella
*/
public void mostrarTabla() {
for(int i = 0; i < 2; i++) {
if(i == 0) {
System.out.print("Tamaño:\t");
} else {
System.out.print("Numero:\t");
}
for(int j = 0; j < mayor; j++) {
System.out.printf("%d\t",tabla[i][j]);
}
System.out.println();
}
}
/**
* Método privado para agregar la cadena que se le pasa como parámetro.
* @param cadena -- Cadena que se agregará a la Lista
*/
private void agregarLista(String cadena) {
cadena = modificaCadena(cadena); // corrige la cadena
palabras.agregar(cadena.length()); // agrega el tamaño de la cadena
if(cadena.length() > mayor) {
mayor = cadena.length(); // asigna el tamaño a la variable mayor
}
}
/**
* Método que modifica una cadena para evitar que se cuenten espacios en
* blanco y signos de puntuación.
* @param cadena -- Cadena a modificar
* @return String -- Cadena modificada
*/
private String modificaCadena(String cadena) {
String nueva = cadena;
nueva = nueva.replace(".", " ");
nueva = nueva.replace(",", " ");
nueva = nueva.replace(";", " ");
nueva = nueva.replace("(", " ");
nueva = nueva.replace(")", " ");
nueva = nueva.trim();
return nueva;
}
/**
* Pruebas de la clase
*/
public static void main(String[] args) {
RegistroPalabras dePrueba = new RegistroPalabras();
File archivo = new File("archivo.txt");
try {
System.out.println("Texto original\n");
dePrueba.leerArchivo(archivo);
System.out.println();
dePrueba.crearTabla();
dePrueba.mostrarTabla();
} catch(IOException ioe) {
System.out.println("Error.");
}
}
}<file_sep>/Caracteres/PruebaCaracteres.java
public class PruebaCaracteres {
public static void main(String[] args) {
Caracteres meeel = new Caracteres();
System.out.println(meeel.revString("ola k ase? %$#$&%$#&//%&)$#W"));
}
}
<file_sep>/Reloj/Reloj.java
import java.util.Date;
/**
* Clase para manejar un reloj con apoyo de la clase Date
* de Java.
* @author <NAME>
* @version 1.00 Junio 2014
*/
public class Reloj {
/**
* Objeto Date para obtener las fechas.
*/
private Date fecha;
/**
* Constructor por omisión.
* Inicializa los atributos en un estado válido.
*/
public Reloj() {
this.fecha = new Date();
}
/**
* Devuelve la hora en Buenos Aires.
* @return Cadena con la hora en Buenos Aires.
*/
public String horaBuenosAires() {
return daHora(3);
}
/**
* Devuelve la hora en Canberra.
* @return Cadena con la hora en Caberra.
*/
public String horaCanberra() {
return daHora(16);
}
/**
* Devuelve la hora en Brazilia.
* @return Cadena con la hora en Brazilia.
*/
public String horaBrazilia() {
return daHora(2);
}
/**
* Devuelve la hora en Otawa.
* @return Cadena con la hora en Otawa.
*/
public String horaOtawa() {
return daHora(2);
}
/**
* Devuelve la hora en Santiado.
* @return Cadena con la hora en Santiago.
*/
public String horaSantiago() {
return daHora(2);
}
/**
* Devuelve la hora en Bogotá.
* @return Cadena con la hora en Bogotá.
*/
public String horaBogota() {
return daHora(1);
}
/**
* Devuelve la hora en La Habana.
* @return Cadena con la hora en La Habana.
*/
public String horaLaHabana() {
return daHora(1);
}
/**
* Devuelve la hora en Madrid.
* @return Cadena con la hora en Madrid.
*/
public String horaMadrid() {
return daHora(7);
}
/**
* Devuelve la hora en París.
* @return Cadena con la hora en París.
*/
public String horaParis() {
return daHora(7);
}
/**
* Devuelve la hora en Tokio.
* @return Cadena con la hora en Tokio.
*/
public String horaTokio() {
return daHora(15);
}
/**
* Devuelve la hora en México D.F.
* @return Cadena con la hora en México D.F.
*/
public String horaMexicoDF() {
return daHora(0);
}
/**
* Devuelve la hora en Nueva York.
* @return Cadena con la hora en Nueva York.
*/
public String horaNuevaYork() {
return daHora(1);
}
private String daHora(int param) {
if (param < 0) // validamos el parámetro
param = 0;
int i = (fecha.getHours() + param) % 24;
String str1 = Integer.toString(i); // obtiene la hora
if (str1.length() == 1) // agrega un 0 para mejorar el formato
str1 = "0" + str1;
String str2 = Integer.toString(fecha.getMinutes()); // obtiene los minutos
if (str2.length() == 1)
str2 = "0" + str2; // mejora el formato
return str1 + ":" + str2 + "hrs"; // regresa la hora con formato
}
} | 0404048e6e73875bebc21a95d9924f94b7824b7b | [
"Java"
] | 24 | Java | manu-msr/java-noob | 9a0ced90796ff72f042e4ce0e069610318c1203d | 7b8dc7c576f6881053f05afb5879e7c168d1d91e | |
refs/heads/master | <file_sep>[](http://ci.testling.com/jameskyburz/testling-test)
[](https://api.travis-ci.org/JamesKyburz/testling-test.png)
<file_sep>var test = require('tape');
test('default test', function(t) {
t.plan(1);
t.equal(1, 1, '1==1');
});
| e259ef58de523d9c707770bf7811ffe819ed27ac | [
"Markdown",
"JavaScript"
] | 2 | Markdown | JamesKyburz/testling-test | c9dd35e3cfae6319a49935855bb553f6f192ccbc | 717106fc78162f4cd03ad5f33af16c2446c4480f | |
refs/heads/master | <repo_name>mjadiaz/BL-SSM<file_sep>/HepAid/submit
#!/bin/bash
#SBATCH --job-name=Scan
#SBATCH --ntasks-per-node=16
#SBATCH --time=4:00:00
source /home/mjad1g20/.bashrc
#module load conda/py3-latest
source activate HepAid
#conda env list
python --version
which python
#python BLSSM.py
#python BLSSM_HEscan_DoublePeak.py
python BLSSM_mg5.py<file_sep>/The-model.md
## [ Double Higgs peak in the minimal SUSY B − L model](https://arxiv.org/pdf/1409.7837.pdf).
We want calculate in the BLSSM model, the processes $h^{\prime} \rightarrow Z Z \rightarrow 4 l$ and $h^{\prime} \rightarrow \gamma \gamma$ with the following benchmark in the low energy scale:
![[LowEnergyParameterPoint.png | 450]]
## Useful resources
- [ Di-photon decay of a light Higgs state in the BLSSM](https://arxiv.org/pdf/2012.04952.pdf)
- [ Exploring new models in all detail with SARAH](https://arxiv.org/pdf/1503.04200.pdf)
- [Mass sprecturm for the minimal SUSY *B-L* model ](https://arxiv.org/pdf/1112.4600.pdf)
- [Beyond-MSSM Higgs sectors](https://arxiv.org/pdf/1409.7182.pdf)
- [The Higgs sector of the minimal SUSY B − L model](https://arxiv.org/pdf/1504.05328.pdf)<file_sep>/Codes/submit_delphes
#!/bin/bash
#SBATCH --job-name=Delphes
#SBATCH --ntasks-per-node=4
module load conda/py3-latest
source activate root_env
python run_delphes.py
<file_sep>/Codes/read_lhe.py
#%%
import pylhe
import math
import numpy as np
import pandas as pd
import os
import subprocess
import gzip
import shutil
from compute_processes import process, madgraph_path, param_card_name, param_card_path, output_name
#%%
#process = [['p p > z,( z > l+ l- a $a,( a > l+ l-))', 'ppza4l_test_2']]
def invariant_mass(p1, p2, p3, p4):
return math.sqrt(
sum(
(1 if mu == "e" else -1) * (getattr(p1, mu) + getattr(p2, mu) + getattr(p3, mu) + getattr(p4, mu)) ** 2
for mu in ["e", "px", "py", "pz"]
)
)
def PT(p1):
return math.sqrt(
sum((getattr(p1, mu)) ** 2 for mu in ["px", "py"])
)
# %%
if __name__ == '__main__':
cut_pt = 5
cut = True
# Create directory for store the data
if not os.path.exists('double_peak_data'):
os.makedirs('double_peak_data')
for p in range(3):
lhe_path = madgraph_path+output_name+process[p][1]+'/Events/run_01'
lhe_file = lhe_path+'/unweighted_events.lhe'
# Unzip the LHE.gz files
with gzip.open(lhe_path+'/unweighted_events.lhe.gz', 'rb') as f_in:
with open(lhe_file, 'wb') as f_out:
shutil.copyfileobj(f_in, f_out)
# Get the invariant mass data for each process
invariant_mass_vec = np.array([]) # Vector to store the invariant mass
for e in pylhe.readLHE(lhe_file):
# block raising an exception
m = invariant_mass(e.particles[-1], e.particles[-2], e.particles[-3], e.particles[-4])
PT_event_list = [PT(e.particles[-1]),PT(e.particles[-2]),PT(e.particles[-3]),PT(e.particles[-4])]
above_cut_test = all(x > cut_pt for x in PT_event_list)
if cut == True:
if above_cut_test == True:
print('all above cut in: ', PT_event_list)
invariant_mass_vec=np.append(invariant_mass_vec,m)
# print('at least one bellow cut in: ', PT_event_list)
# print(invariant_mass_vec)
else:
invariant_mass_vec=np.append(invariant_mass_vec,m)
df = pd.DataFrame(invariant_mass_vec,columns=['imass'])
print(df.head(), len(df))
df.to_csv('double_peak_data/'+process[p][1]+'.csv')
print(lhe_path, ' Done')
<file_sep>/README.md
### Model Files
The Sarah file was taken from [B-L-SSM – Trac – Hepforge (sarah.hepforge.org)](https://sarah.hepforge.org/trac/wiki/B-L-SSM). This model was created by L.Basso, F.Staub. The UFO and SPHENO files where created in Mathematica by SARAH by an `generate_model.m` through:
```mathematica
Needs["SARAH`","/mainfs/home/mjad1g20/HEP/SARAH/SARAH-4.14.5/SARAH.m"]
Start["B-L-SSM"]
ModelOutput[EWSB]
MakeSPheno[]
MakeUFO[]
MakeCHep[]
Quit[];
```
- There is an option to [create the CalcHep](https://gitlab.in2p3.fr/goodsell/sarah/-/wikis/CalcHep/CompHep) file to run it with spheno, that might be helpful.
- `RunSPhenoViaCalcHep`: writes C code to run SPhenofrom the graphical interface of CalcHepto calculate the spectrum on the fly.
- There are more options that should be looked.
#### Useful links
- [Running Jupyter Notebook on a remote server](https://docs.anaconda.com/anaconda/user-guide/tasks/remote-jupyter-notebook/)
<file_sep>/Codes/pySpheno.py
import os
import re
import subprocess
import time
class pySpheno:
def __init__(self, spheno_dir):
self._dir=spheno_dir
self.models = pySpheno._models_in_dir(self)
def _models_in_dir(self):
models = []
for f in os.listdir(self._dir+'/bin'):
if not (re.search(r'SPheno\w+',f)==None):
m = re.search('(?<=SPheno).*','SPhenoBLSSM')[0]
models.append(m)
return models
def run(self, model, in_file, out_file):
#subprocess.call([self._dir+'/bin'+'/SPheno'+model, in_file])
run = subprocess.run([self._dir+'/bin'+'/SPheno'+model, in_file, out_file], capture_output=True, text=True)
if 'Finished' in run.stdout:
print(run.stdout)
else:
print('Error')
spheno_dir = '/home/mjad1g20/HEP/SPHENO/SPheno-3.3.8'
in_file = '/mainfs/home/mjad1g20/HEP/SPHENO/SPheno-3.3.8/LesHouches.in.BLSSM_low'
out_file = '/mainfs/home/mjad1g20/HEP/WorkArea/BLSSM-Double-Peak/Codes/spheno_cards/param_card.dat'
spheno = pySpheno(spheno_dir)
spheno.run(model='BLSSM', in_file=in_file, out_file=out_file)
<file_sep>/Codes/submit
#!/bin/bash
#SBATCH --job-name=MadRun
#SBATCH --ntasks-per-node=16
module load conda/py3-latest
#conda env list
source activate root_env
python --version
python compute_processes.py
<file_sep>/Codes/run_delphes.py
import os
import subprocess
import gzip
import shutil
#from compute_processes import process, output_name, madgraph_path
process= [['p p > z > l+ l- a $a , a > l+ l-', 'ppza4l'],
['p p > h1 > z z, (z > l+ l-, z > l+ l-)', 'pph14l'],
['p p > h2 > z z, (z > l+ l-, z > l+ l-)', 'pph24l'],
['p p > h1 > a a','pph1aa'],
['p p > h2 > a a','pph2aa']]
madgraph_path = '/home/mjad1g20/HEP/MG5/MG5_aMC_v3_1_1'
output_name = '/double_peak/'
delphes_path = '/home/mjad1g20/HEP/DELPHES/Delphes-3.5.0'
delphes_card = '/mainfs/home/mjad1g20/HEP/DELPHES/Delphes-3.5.0/cards/delphes_card_CMS.tcl'
DelphesHepMC2 = '/mainfs/home/mjad1g20/HEP/DELPHES/Delphes-3.5.0/DelphesHepMC2'
#print(DelphesHepMC2)
if not os.path.exists(delphes_path+output_name):
print('Create directory for Delphes output: {}'.format(output_name))
os.makedirs(delphes_path+output_name)
def gunzip(gzfile, gunzipfile):
with gzip.open(gzfile, 'rb') as f_in:
with open(gunzipfile, 'wb') as f_out:
shutil.copyfileobj(f_in, f_out)
for p in process:
_, p_name = p
hepmc_path = madgraph_path+output_name+p_name+'/Events/run_01/tag_1_pythia8_events.hepmc'
delphes_output = delphes_path+output_name+p_name+'.root'
print(hepmc_path)
if os.path.exists(delphes_output):
print('Removing existing .root output for the process')
os.remove(delphes_output)
if not os.path.exists(hepmc_path):
print('Unzip hepmc.gz for DelphesHepMC2')
gunzip(hepmc_path+'.gz',hepmc_path)
print('Running DelphesHepMC2')
#run_delphes = subprocess.run([DelphesHepMC2, delphes_card, delphes_output, hepmc_path], capture_output=True, text=True)
subprocess.run([DelphesHepMC2, delphes_card, delphes_output, hepmc_path])
# if os.path.exists(delphes_path+output_name+p_name+'_output.txt'):
# os.remove(delphes_path+output_name+p_name+'_output.txt')
# with open(delphes_path+output_name+p_name+'_output.txt','w') as f:
# f.write(run_delphes.stdout)
# f.write(run_delphes.stderr)
print('Removing hepmc file ... t0o heavy')
os.remove(hepmc_path)
<file_sep>/HepAid/BLSSM_mg5.py
import HepRead
import HepTools
import os
model = 'BLSSM'
work_dir = '/scratch/mjad1g20/HEP/WorkArea/BLSSM_Work'
spheno_dir = '/scratch/mjad1g20/HEP/SPHENO/SPheno-3.3.8'
reference_lhs = '/scratch/mjad1g20/HEP/SPHENO/SPheno-3.3.8/BLSSM/Input_Files/LesHouches.in.BLSSM'
madgraph_dir = '/scratch/mjad1g20/HEP/MG5_aMC_v3_1_1'
# Initiating Madgraph
mg5 = HepTools.Madgraph(madgraph_dir, work_dir)
# Selected param_card.dat
param_card_path = os.path.join(work_dir,'SPhenoBLSSM_output/SPheno.spc.BLSSM_HEscan_770')
# UFO model name in madraph_dir
ufo_model = 'BLSSM-2'
# Creating the Madgraph script
script = HepRead.MG5Script(work_dir,ufo_model)
processes = [['p p > h1, h1 > a a', 'pph1aa'],
['p p > h2, h2 > a a', 'pph2aa'],
['p p > a a / h1 h2', 'ppaa_bg']]
for p in processes:
process, syntax = p
script.process(process)
script.output(syntax)
script.launch(syntax)
script.param_card(param_card_path)
script.delphes_card('/mainfs/scratch/mjad1g20/HEP/MG5_aMC_v3_1_1/Delphes/cards/delphes_card_CMS.tcl')
script.write()
mg5.run()
<file_sep>/HepAid/HepTools.py
import os
import re
import subprocess
from scipy.stats import loguniform
import matplotlib.pyplot as plt
import numpy as np
import HepRead
class Spheno:
'''
## Spheno class
- Identifies what models are available in the work direction. \n
and store all the models in .model_list \n
- Call .run(in, out) to run Spheno with input and output files. \n
The output file will be stored in a folder called SPhenoModel_output \n
inside work_dir.
Todo:
- Figure out how to redirect the additional files that spheno creates in a run.
'''
def __init__(self, spheno_dir, work_dir, model=None, input_lhs=None):
self._dir=spheno_dir
self.model_list = Spheno._models_in_dir(self)
self._model = Spheno._model_init(self, model)
self.work_dir = work_dir
def _model_init(self, model):
if model == None:
if len(self.model_list) == 1:
return self.model_list[0]
else:
return print('Define model. \nModels available: {}'.format(self.model_list))
else:
print(f'{model} model activated.')
return model
def _models_in_dir(self):
models_in_dir = []
for f in os.listdir(self._dir+'/bin'):
if not (re.search(r'SPheno\w+',f)==None):
m = re.search('(?<=SPheno).*',f)[0]
models_in_dir.append(m)
return models_in_dir
def run(self, in_file_name, out_file_name, mode='local'):
out_dir = os.path.join(self.work_dir, 'SPheno'+self._model+'_output')
in_file = os.path.join(self.work_dir, 'SPheno'+self._model+'_input',in_file_name)
if not(os.path.exists(out_dir)):
os.makedirs(out_dir)
file_dir=os.path.join(out_dir,out_file_name)
print(f'Save {out_file_name} in :{file_dir}')
if mode == 'local':
run = subprocess.run([self._dir+'/bin'+'/SPheno'+self._model, in_file, file_dir], capture_output=True, text=True)
if 'Finished' in run.stdout:
print(run.stdout)
return file_dir
else:
print('Parameters point error!')
print(run.stdout)
return None
elif mode == 'cluster':
print('Implement cluster mode')
class Scanner:
'''
Class containing useful tools to do scans with SPheno and Madgraph.
Maybe it will a bunch of functions.
To do:
- Implement general scanner function (for loops)
- How to store these functions Class or what...
'''
def rlog_array(min, max, n_points, show=False):
'''
Creates a random array distributed uniformly in log scale. \n
- from min to max with n_points number of points. \n
- turn show=True to see the histogram of the distribution.
'''
ar = loguniform.rvs(min, max, size=n_points)
if show == True:
plt.hist(np.log10(ar),density=True, histtype='step',color='orange')
plt.hist(np.log10(ar),density=True, histtype='stepfilled', alpha=0.2, color='orange')
plt.xlabel('Variable [log]')
plt.show()
return ar
def rlog_float(min, max):
'''
Creates a random float distributed uniformly in log scale.
'''
ar = loguniform.rvs(min, max, size=1)
return ar[0]
def runiform_array(min,max, n_points, show=False):
'''
Create a uniform random array from min to mas with n_points.
- show= True to see the distribution histogram.
'''
ar=np.random.uniform(min, max ,size = n_points)
if show == True:
plt.hist(ar,density=True, histtype='step',color='orange')
plt.hist(ar,density=True, histtype='stepfilled', alpha=0.2, color='orange')
plt.xlabel('Variable')
plt.show()
return ar
def runiform_float(min,max):
'''
Create a uniform random float within min and max.
'''
ar=np.random.uniform(min, max ,size = 1)
return ar[0]
class Madgraph:
'''
Basicaly just run madgraph with in the script mode with the scripts created \n
by the MG5Script class. \n
Todo:
- Figure out how to print some output from madgraph.
'''
def __init__(self, madgraph_dir, work_dir):
self._dir = madgraph_dir
self.work_dir = work_dir
def run(self, input_file = 'MG5Script.txt', mode='local'):
'''
Run madgraph with an script named MG5Script.txt (created by the MG5Script class) in within work_dir. \n
Change input_file to change to another script within work_dir.
'''
if mode == 'local':
subprocess.run([os.path.join(self._dir,'bin/mg5_aMC'), os.path.join(self.work_dir,input_file)])
elif mode == 'cluster':
print('Implement cluster mode.')
class HiggsBounds:
def __init__(self, higgs_bounds_dir, work_dir, model=None, neutral_higgs=None, charged_higgs=None):
self._dir = higgs_bounds_dir
self.work_dir = work_dir
self.model = model
self.neutral_higgs = neutral_higgs
self.charged_higgs = charged_higgs
def run(self):
'''
Runs HiggsBounds with the last point calculated by SPheno. Returns the path for HiggsBounds_results.dat if SPheno and HiggsBounds succeed. If there is an Error in one them it returns None and prints the error output.
'''
run = subprocess.run([os.path.join(self._dir, 'HiggsBounds'), 'LandH', 'effC', str(self.neutral_higgs), str(self.charged_higgs), self.work_dir+'/'], capture_output=True, text=True)
if 'finished' in run.stdout:
print(run.stdout)
return os.path.join(self.work_dir, 'HiggsBounds_results.dat')
else:
print('HiggsBound not finished!')
print(run.stdout)
return None
<file_sep>/Codes/events_invariant_mass_plot.py
#%%
import seaborn as sns # for data visualization
import pandas as pd # for data analysis
import matplotlib.pyplot as plt # for data visualization
import numpy as np
from compute_processes import process, process_1, madgraph_path, param_card_name, param_card_path, output_name
# %%
sns.set(style='darkgrid',)
sns.__version__
# %%
# df_mass1 = pd.read_csv('double_peak_data/'+process[0][1]+'.csv')
# print(process[0][1])
df_mass1 = pd.read_csv('double_peak_data/'+process_1[0][1]+'.csv')
print(process_1[0][1])
df_mass2 = pd.read_csv('double_peak_data/'+process[1][1]+'.csv')
print(process[1][1])
df_mass3 = pd.read_csv('double_peak_data/'+process[2][1]+'.csv')
print(process[2][1])
#%%
ppza4l = df_mass1.imass.to_numpy()
pph14l = df_mass2.imass.to_numpy()
pph24l = df_mass3.imass.to_numpy()
pph14l_vec = [r'$pp\rightarrow h1 \rightarrow ZZ \rightarrow 4l$']*len(pph14l)
pph24l_vec = [r'$pp \rightarrow h2 \rightarrow ZZ \rightarrow 4l$']*len(pph24l)
ppza4l_vec = [r'$pp \rightarrow Z \rightarrow 2l\gamma * \rightarrow 4l$']*len(ppza4l)
df1 = pd.DataFrame(dict(m = pph14l, channel = pph14l_vec), columns=['m', 'channel'])
df2 = pd.DataFrame(dict(m = pph24l, channel = pph24l_vec), columns=['m', 'channel'])
df3 = pd.DataFrame(dict(m = ppza4l, channel = ppza4l_vec), columns=['m', 'channel'])
df = pd.concat([df1,df2,df3])
df
# %%
sns.histplot(
df, x="m", hue="channel", element="step",
)
plt.xlim(0,200)
#plt.yscale('log')
#plt.xlabel(r'$M_{\mu^{+}\mu^{-}} $')
plt.xlabel(r'$M_{4ll} $')
plt.ylabel('Events')
plt.show()
# %%
# %%
<file_sep>/HepAid/HepRead.py
import re
import os
from shutil import copy
import awkward as ak
import numpy as np
from tabulate import tabulate
#########################################
# Classes for reading LesHouches files. #
# Focusing on Spheno. #
#########################################
class BlockLine:
'''## Line:
Each line can be of a different category:
- block_header
- on_off
- value
- matrix_value '''
def __init__(self, entries, line_category):
self.entries = entries
self.line_category = line_category
self.line_format = BlockLine.fline(line_category)
def fline(cat):
''' Text format of each line'''
if cat == 'block_header':
line_format = '{:6s} {:20s} {:13s}'
elif cat == 'on_off':
line_format = '{:6s} {:18s} {:13s}'
elif cat == 'value':
line_format = '{:6s} {:18s} {:13s}'
elif cat == 'matrix_value':
line_format = '{:3s}{:3s} {:18s} {:13s}'
return line_format
class Block:
'''
## Block
It holds each line of a block.\n
Call .show() to print a block. \n
Call .set(parameter_number, value) to change the parameter value in the instance.
'''
def __init__(self, block_name, block_comment=None, category=None):
self.block_name = block_name
self.block_comment = block_comment
self.block_body = []
self.category = category
def show(self):
'''
Print block information in the LesHouches format.
'''
print('{} {} {:10s}'.format('Block',self.block_name,self.block_comment))
for b in self.block_body:
print(b.line_format.format(*b.entries))
def set(self,option, param_value):
'''
Call set(option, param_value) method to modify the option N with a parameter_value \n.
-option = Can be an int or a list [i, j]. \n
-param_value = Can be an int (on/off) or a float. \n
'''
for line in self.block_body:
if (line.line_category == 'matrix_value'):
if (option == [int(line.entries[0]),int(line.entries[1])]):
line.entries[2] = '{:E}'.format(param_value)
print('{} setted to : {}'.format(line.entries[-1], line.entries[1]))
break
if (line.line_category == 'value') & (option == int(line.entries[0])):
line.entries[1] = '{:E}'.format(param_value)
print('{} setted to : {}'.format(line.entries[-1], line.entries[1]))
break
elif (line.line_category == 'on_off') & (option == int(line.entries[0])):
if isinstance(param_value, int):
line.entries[1] = '{}'.format(param_value)
print('{} setted to : {}'.format(line.entries[-1], line.entries[1]))
else:
print('param_value={} is not integer'.format(param_value))
break
class LesHouches:
'''
## LesHuches
Read a LesHouches file and stores each block in block classes. \n
- To get all the names of the blocks call .block_list. \n
- work_dir is the directory where all the outputs will be saved. \n
- The new LesHouches files will be saved in a folder called SPhenoMODEL_input since is the input for spheno.
'''
def __init__(self, file_dir, work_dir, model):
self.file_dir = file_dir
print(f'Reading LesHouches from : {file_dir}')
self._blocks = LesHouches.read_leshouches(file_dir)
self.block_list = [name.block_name for name in self._blocks]
self.work_dir = work_dir
self.model = model
def block(self, name):
block = LesHouches.find_block(name.upper(), self._blocks)
return block
def find_block(name, block_list):
try:
if isinstance(name, str):
for b in block_list:
if b.block_name == name:
b_found = b
break
else:
None
return b_found
except:
print('block not found')
def read_leshouches(file_dir):
block_list = []
paterns = dict( block_header= r'(?P<block>BLOCK)\s+(?P<block_name>\w+)\s+(?P<comment>#.*)',
on_off= r'(?P<index>\d+)\s+(?P<on_off>-?\d+\.?)\s+(?P<comment>#.*)',
value= r'(?P<index>\d+)\s+(?P<value>-?\d+\.\d+E.\d+)\s+(?P<comment>#.*)',
matrix_value= r'(?P<i>\d+)\s+(?P<j>\d+)\s+(?P<value>-?\d+\.\d+E.\d+)\s+(?P<comment>#.*)')
with open(file_dir, 'r') as f:
for line in f:
# Match in 3 groups a pattern like: '1000001 2.00706278E+02 # Sd_1'
m_block = re.match(paterns['block_header'], line.upper().strip())
if not(m_block == None):
if m_block.group('block_name') in ['MODSEL','SPHENOINPUT','DECAYOPTIONS']:
block_list.append(Block( block_name=m_block.group('block_name'),
block_comment=m_block.group('comment'),
category= 'spheno_data' ))
in_block, block_from = m_block.group('block_name'), 'spheno_data'
else:
block_list.append(Block( block_name=m_block.group('block_name'),
block_comment=m_block.group('comment'),
category= 'parameters_data'))
in_block, block_from = m_block.group('block_name'), 'parameters_data'
m_body = re.match(paterns['on_off'], line.strip())
if not(m_body == None):
LesHouches.find_block(in_block,block_list).block_body.append(BlockLine(list(m_body.groups()),'on_off'))
m_body = re.match(paterns['value'], line.strip())
if not(m_body == None):
LesHouches.find_block(in_block,block_list).block_body.append(BlockLine(list(m_body.groups()),'value'))
m_body = re.match(paterns['matrix_value'], line.strip())
if not(m_body == None):
LesHouches.find_block(in_block,block_list).block_body.append(BlockLine(list(m_body.groups()), 'matrix_value'))
return block_list
def new_file(self, new_file_name):
'''
Writes a new LesHouches file with the blocks defined in the instance. \n
Possibly with new values for the parameters and options.
'''
new_file_dir = os.path.join(self.work_dir, 'SPheno'+self.model+'_input')
if not(os.path.exists(new_file_dir)):
os.makedirs(new_file_dir)
file_dir=os.path.join(new_file_dir,new_file_name)
print(f'Writing new LesHouches in :{file_dir}')
with open(file_dir,'w+') as f:
for block in self._blocks:
head = '{} {} {:10s}'.format('Block',block.block_name,block.block_comment)+'\n'
f.write(head)
for b in block.block_body:
f.write(b.line_format.format(*b.entries)+'\n')
###################################
# Classes for reading SLHA files. #
# Focusing on madgraph. #
###################################
class Particle():
def __init__(self, pid, ufo_name=None, total_width=None, mass=None, comment=None, decays=None):
self.pid = pid
self._ufo_name = ufo_name # To link it with madgraph but maybe it is not necessary.
self.total_width = total_width
self.mass = mass
self.comment = comment
# How to store the decays
self.decays = decays
def find_particle(id, particles_list):
try:
if isinstance(id, int):
for p in particles_list:
if p.pid == id:
p_found = p
break
else:
None
elif isinstance(id, str):
for p in particles_list:
if p.comment == id:
p_found = p
break
else:
None
return p_found
except:
print('No particle found')
def show(self):
#particle = Particle.find_particle(pid, particles_list)
n_data = lambda n: [self.decays[n][i] for i in self.decays.fields]
n_decays = ak.num(self.decays,axis=0)
data = [n_data(n) for n in range(n_decays)]
headers=[field.upper() for field in self.decays.fields]
print(tabulate([[self.mass,self.total_width]], headers=['MASS GeV', "TOTAL WIDTH GeV"],tablefmt="fancy_grid"))
print(tabulate(data, headers=headers,tablefmt="fancy_grid"))
class Slha:
'''
## Read SLHA
Read the given model file in SLHA format. It stores PID of all the particles of the models in .model_particles \n
in a list of tuples (PID, NAME) to have a sense of what particles are in the model. \n
The internal list _particles contain each particle saved as a Particle class so that we can use \n
all the internal methods and properties of this class, for example:
- .particle('25').show() ---> It display all the information about the particle.
- .particle('25').mass
\n
This class focuses on extracting information not writing the file because we always can \n
change the parameters inside madgraph by the command: set parameter = value.
'''
def __init__(self, model_file):
self.model_file = model_file
self._particles, self.model_particles = Slha.read_file(model_file)
def read_file(model_file):
'''
Read all the particles of the model saving it in the particles object list. \n
It creates a Particle class for each particle of the model reading it \n
from the MASS block of the SLHA file.
'''
with open(model_file, 'r') as f:
slha_file = f.read() # Read the whole file
slha_blocks = re.split('BLOCK|Block|block|DECAY|Decay|decay', slha_file) # Separate the text file into parameter blocks and decay blocks
particles = [] # Initiate the Partcle objects list.
for block in slha_blocks:
block = re.split('\n',block) # Split block in lines
# Create the particles of the model from the MASS block and populate the
# particles list with Particles objects.
if "MASS" in block[0].split()[0].upper():
for line in block:
line = re.split('\s+',line.strip())
#print(l)
if line[0].isnumeric(): # To skip to the first particle information, since the first element is the PID (numeric)
particles.append(Particle(int(line[0]))) # Append to particles list a Particle object with the PID given by l[0]
particles[-1].mass = float(line[1]) # Assign the mass for the given Particle object
particles[-1].comment = line[3] # Assign the ufo_name wich is the comment (last element of the line).
# Note: Not always is the ufo_name.
#print(particles[-1].pid, particles[-1].mass, particles[-1].comment) # Just to check
break
# Another foor loop because we want to create the particles first
for block in slha_blocks:
block = re.split('\n',block) # Split block in lines again
# If the first element on the first line is numeric correspond to decay
# block with that ID value else is a parameter block.
if block[0].split()[0].isnumeric():
tmp_pid = int(block[0].split()[0]) # Temporal particle id for a decay block
decays = ak.ArrayBuilder() # Initiate the decays as an awkward array builder for the current block
for line in block:
# Match in 3 groups a pattern like: '1000001 2.00706278E+02 # Sd_1'
match = re.match(r'(?P<pid>\d+)\s+(?P<width>\d+\.\d+E.\d+)\s+#(?P<comment>.*)', line.strip())
if not(match == None):
#print('PID: {}, Width: {:E}, Comment: {}'.format(int(match.group('pid')),float(match.group('width')) ,match.group("comment").strip()))
Particle.find_particle(tmp_pid,particles).total_width = float(match.group('width'))
#print(Particle.find_particle(tmp_pid,particles).pid, Particle.find_particle(tmp_pid,particles).total_width)
# Match in 4 groups a pattern like: '2.36724485E-01 2 2 -1000024 # BR(Sd_1 -> Fu_1 Cha_1 )'
match = re.match(r'(?P<br>\d+\.\d+E.\d+)\s+(?P<nda>\d+)\s+(?P<fps>.*?)\s+#(?P<comment>.*)', line.strip())
if not(match == None):
tmp_decay = ak.Array([{ "br": float(match.group('br')),
"nda": int(match.group('nda')),
"fps": [int(p) for p in match.group('fps').split()],
"comment": match.group('comment').strip()}])
decays.append(tmp_decay, at=0)
#print(tmp_decay)
Particle.find_particle(tmp_pid,particles).decays = decays.snapshot()
def particle_ids(particles_list):
''' Returns a simple list holding all the particle ID of the model'''
pid_list=[(p.pid, p.comment) for p in particles_list]
#comment_list = [p.comment for p in particles_list]
#pid_list.sort()
return pid_list
particles_list = particle_ids(particles)
return particles, particles_list
def particle(self, pid):
particle = Particle.find_particle(pid, self._particles)
return particle
#######################################
# Class for writing a Madgraph script #
#######################################
class MG5Script:
'''
Create a object containing mostly* all the necesary commands to compute a process in madgraph with the \n
text-file-input/script mode. A default or template input file can be preview with the show() method within this class.
'''
def __init__(self,work_dir, ufo_model):
self.work_dir = work_dir
self.ufo_model = ufo_model
self._default_input_file()
def import_model(self):
out = 'import model {} --modelname'.format(self.ufo_model)
self._import_model = out
def define_multiparticles(self,syntax = ['p = g d1 d2 u1 u2 d1bar d2bar u1bar u2bar', 'l+ = e1bar e2bar', 'l- = e1 e2']):
out = []
if not(syntax==None):
[out.append('define {}'.format(i)) for i in syntax]
self._define_multiparticles = out
else:
self._define_multiparticles = None
def process(self,syntax = 'p p > h1 > a a'):
'''
Example: InputFile.process('p p > h1 > a a')
'''
out = 'generate {}'.format(syntax)
self._process = out
def add_process(self,syntax = None):
'''
Example: InputFile.add_process('p p > h2 > a a')
'''
out = []
if not(syntax==None):
[out.append('add process {}'.format(i)) for i in syntax]
self._add_process= out
else:
self._add_process= None
def output(self, name='pph1aa'):
output_dir = os.path.join(self.work_dir,name)
out = 'output {}'.format(output_dir)
self._output = out
def launch(self, name='pph1aa'):
launch_dir = os.path.join(self.work_dir,name)
out = 'launch {}'.format(launch_dir)
self._launch = out
def shower(self,shower='Pythia8'):
'''
Call .shower('OFF') to deactivate shower effects.
'''
out ='shower={}'.format(shower)
self._shower = out
def detector(self,detector='Delphes'):
'''
Call .detector('OFF') to deactivate detector effects.
'''
out ='detector={}'.format(detector)
self._detector = out
def param_card(self, path=None):
if path==None:
self._param_card = None
else:
self._param_card = path
def delphes_card(self, path=None):
if path==None:
self._delphes_card = None
else:
self._delphes_card = path
def set_parameters(self,set_param=None):
if set_param==None:
self._set_parameters = None
else:
out = ['set {}'.format(i) for i in set_param]
self._set_parameters = out
def _default_input_file(self):
self.import_model()
self.define_multiparticles()
self.process()
self.add_process()
self.output()
self.launch()
self.shower()
self.detector()
self.param_card()
self.delphes_card()
self.set_parameters()
def show(self):
'''
Print the current MG5InputFile
'''
write = [self._import_model, self._define_multiparticles, self._process, self._add_process, self._output, self._launch, self._shower,
self._detector, '0', self._param_card, self._delphes_card, self._set_parameters, '0']
for w in write:
if not(w==None):
if isinstance(w,str):
print(w)
elif isinstance(w,list):
[print(i) for i in w]
def write(self):
'''
Write a new madgraph script as MG5Script.txt used internally by madgraph.
'''
write = [self._import_model, self._define_multiparticles, self._process, self._add_process, self._output, self._launch, self._shower,
self._detector, '0', self._param_card, self._delphes_card, self._set_parameters, '0']
f = open(os.path.join(self.work_dir,'MG5Script.txt'),'w+')
for w in write:
if not(w==None):
if isinstance(w,str):
f.write(w+'\n')
elif isinstance(w,list):
[f.write(i+'\n') for i in w]
f.close()
return
##########################################
# Class for reading a HiggsBounds output #
##########################################
class HiggsBoundsResults:
def __init__(self, work_dir, model=None):
self.work_dir = work_dir
self.model = model
def read(self, direct_path=None):
'''
Read HiggsBounds_results.dat and outputs a tuple with: \n
(HBresult, chan, obsratio, ncomb)
'''
if direct_path:
file_path = direct_path
else:
file_path = os.path.join(self.work_dir, 'HiggsBounds_results.dat')
with open(file_path , 'r') as f:
for line in f:
line = line.strip()
if ('HBresult' in line) & ('chan' in line):
line = line.split()[1:]
index_hbresult = line.index('HBresult')
index_chan = line.index('chan')
index_obsratio = line.index('obsratio')
index_ncomb = line.index('ncomb')
#print(line)
_ = f.readline()
for subline in f:
subline = subline.split()
#print(subline[index_hbresult],subline[index_chan],subline[index_obsratio],subline[index_ncomb])
break
return (subline[index_hbresult],subline[index_chan],subline[index_obsratio],subline[index_ncomb])
def save(self, output_name,in_spheno_output = True):
if in_spheno_output:
copy(os.path.join(self.work_dir, 'HiggsBounds_results.dat'), os.path.join(self.work_dir,'SPheno'+self.model+'_output' ,'HiggsBounds_results_'+str(output_name)+'.dat'))
pass
<file_sep>/Codes/compute_processes.py
#%%
import os
import subprocess
import random
#%%
############################
# Paths and processes info #
############################
# MadGraph process syntax and launch/output name
#process= [['p p > h1 > z z, z > l+ l-,z > l+ l-', 'pph12z4l']]
#process= [['p p > h2 > a a', 'pph2aa']]
process= [['p p > h1 > a a','pph1aa'],
['p p > h2 > a a','pph2aa'],
['p p > h1 > z z, (z > l+ l-, z > l+ l-)', 'pph14l'],
['p p > h2 > z z, (z > l+ l-, z > l+ l-)', 'pph24l'],
['p p > z > l+ l- a $a , a > l+ l-', 'ppza4l']]
# wget https://launchpad.net/mg5amcnlo/3.0/3.1.x/+download/MG5_aMC_v3.1.1.tar.gz
madgraph_path = '/scratch/mjad1g20/HEP/MG5_aMC_v3_1_1'
param_card_name_2 = '/SPheno.spc.BLSSM'
param_card_path_2 = '/home/mjad1g20/HEP/SPHENO/SPheno-3.3.8'
param_card_name = '/param_card.dat'
param_card_path = '/home/mjad1g20/HEP/WorkArea/BLSSM-Double-Peak/Codes/Cards'
delphes_card = '/mainfs/home/mjad1g20/HEP/WorkArea/BLSSM-Double-Peak/Codes/Cards/delphes_card_CMS.dat'
output_name = '/double_peak/'
#################################
# Compute processes in Madgraph #
#################################
if __name__ == '__main__':
#for p in range(len(process)):
for p in process:
mg5_syntax, p_name = p
f = open(madgraph_path+'/compute_process.txt',"w+")
f.write('import model BLSSM-2 --modelname\n')
f.write('define p = g d1 d2 u1 u2 d1bar d2bar u1bar u2bar\n')
f.write('define l+ = e1bar e2bar\n')
f.write('define l- = e1 e2\n')
f.write('generate '+mg5_syntax+'\n')
while os.path.exists(madgraph_path+output_name+p_name):
p_name = p_name+'_'+str(random.randint(1,100))
f.write('output '+madgraph_path+output_name+p_name+'\n')
f.write('launch '+madgraph_path+output_name+p_name+'\n')
f.write('shower=Pythia8\n')
f.write('detector=Delphes\n')
f.write('0\n')
f.write(param_card_path_2+param_card_name_2+'\n')
f.write(delphes_card+'\n')
f.write('set mh1 = 125\n')
f.write('set mh2 = 136\n')
f.write('set ebeam1 = 4000.0\n')
f.write('set ebeam2 = 4000.0\n')
f.write('0\n')
f.close()
subprocess.call([madgraph_path+'/bin/mg5_aMC', madgraph_path+'/compute_process.txt'])
break
os.remove(madgraph_path+'/compute_process.txt')
<file_sep>/HepAid/BLSSM_HEscan.py
import numpy as np
import HepRead
import HepTools
import os
from HepTools import Scanner as sc
model = 'BLSSM'
work_dir = '/scratch/mjad1g20/HEP/WorkArea/BLSSM_Work'
spheno_dir = '/scratch/mjad1g20/HEP/SPHENO/SPheno-3.3.8'
reference_lhs = '/scratch/mjad1g20/HEP/SPHENO/SPheno-3.3.8/BLSSM/Input_Files/LesHouches.in.BLSSM'
madgraph_dir = '/scratch/mjad1g20/HEP/MG5_aMC_v3_1_1'
SPhenoBLSSM = HepTools.Spheno(spheno_dir, work_dir ,model)
lhs = HepRead.LesHouches(reference_lhs, work_dir, model)
#print('Blocks in High Energy LesHouches:')
#print('---------------------------------')
#print(lhs.block_list)
#lhs.block('MODSEL').show()
#lhs.block('SMINPUTS').show()
# Fixed parameters
lhs.block('MINPAR').set(8,2500)
lhs.block('MINPAR').set(7,1.15)
lhs.block('MINPAR').set(3,5)
lhs.block('MINPAR').show()
[lhs.block('extpar').set(i,0) for i in [11,12,13,14]]
lhs.block('extpar').set(3,2500)
lhs.block('extpar').set(10,1.15)
lhs.block('extpar').set(1,0.55)
lhs.block('extpar').set(2,-0.12)
lhs.block('extpar').show()
option = [13, 16,78,520,67,60]
value = [3,0,1,0,1,1]
for i,j in zip(option, value):
lhs.block('sphenoinput').set(i,j)
#lhs.block('sphenoinput').show()
option = [1,2,3,4,5,6,7,8,9,1001,1002,1003,1004,1005,1006,1007,1008,1009,1010,1011,1012,1013]
value = np.zeros(len(option),dtype = int)
for i,j in zip(option, value):
lhs.block('decayoptions').set(i,int(j))
#lhs.block('decayoptions').show()
# Scanned parameters
m0 = lambda low, high: sc.runiform_float(low,high)
m12 = lambda low, high: sc.runiform_float(low,high)
a0 = lambda low, high: sc.runiform_float(low,high)
tanbeta = lambda low, high: sc.runiform_float(low,high)
N_POINTS = 1300
points = 0
index = []
for i in range(N_POINTS):
lhs.block('MINPAR').set(1,m0(100,1000))
lhs.block('MINPAR').set(2,m12(1000,4500))
lhs.block('MINPAR').set(5,a0(1000,4000))
lhs.block('MINPAR').set(3,tanbeta(1,60))
new_lhs_name = 'LesHouches.in.BLSSM_HEscan_'+str(i)
out_spheno_name = 'SPheno.spc.BLSSM_HEscan_'+str(i)
# Creating the new LesHouches file, and runing Spheno.
lhs.new_file(new_lhs_name)
param_card_path = SPhenoBLSSM.run(new_lhs_name,out_spheno_name)
# Reading spheno output
if not(param_card_path==None):
points = points + 1
index.append(i)
with open('number_of_points.txt','w+') as f:
f.write(f'{points} \n')
for i in index:
f.write(f'{i} \n')
<file_sep>/HepAid/BLSSM_HEscan_DoublePeak.py
import numpy as np
import HepRead
import HepTools
import os
from HepTools import Scanner as sc
model = 'BLSSM'
work_dir = '/scratch/mjad1g20/HEP/WorkArea/BLSSM_DoublePeak'
spheno_dir = '/scratch/mjad1g20/HEP/SPHENO/SPheno-3.3.8'
reference_lhs = '/scratch/mjad1g20/HEP/SPHENO/SPheno-3.3.8/BLSSM/Input_Files/LesHouches.in.BLSSM'
madgraph_dir = '/scratch/mjad1g20/HEP/MG5_aMC_v3_1_1'
SPhenoBLSSM = HepTools.Spheno(spheno_dir, work_dir ,model)
lhs = HepRead.LesHouches(reference_lhs, work_dir, model)
# Fixed parameters
option, value = [8,7,3], [1700,1.15,5]
for i,j in zip(option, value):
lhs.block('MINPAR').set(i,j)
[lhs.block('EXTPAR').set(i,0) for i in [11,12,13,14]]
option, value = [3,10,1,2], [1700,1.15,0.55,-0.12]
for i,j in zip(option, value):
lhs.block('EXTPAR').set(i,j)
# Spheno options to run with Madgraph
option = [13, 16,78,520,67,60]
value = [3,0,1,0,1,1]
for i,j in zip(option, value):
lhs.block('sphenoinput').set(i,j)
# Turning off loop decay options because they are already defined
option = [1,2,3,4,5,6,7,8,9,1001,1002,1003,1004,1005,1006,1007,1008,1009,1010,1011,1012,1013]
value = np.zeros(len(option),dtype = int)
for i,j in zip(option, value):
lhs.block('decayoptions').set(i,int(j))
# Scanned parameters
m0 = lambda low, high: sc.runiform_float(low,high)
m12 = lambda low, high: sc.runiform_float(low,high)
a0 = lambda low, high: sc.runiform_float(low,high)
N_POINTS = 1000
points = 0
index = []
for i in range(N_POINTS):
lhs.block('MINPAR').set(1,m0(100,1000))
lhs.block('MINPAR').set(2,m12(2000,4500))
lhs.block('MINPAR').set(5,- a0(1000,4000))
new_lhs_name = 'LesHouches.in.BLSSM_HEscan_'+str(i)
out_spheno_name = 'SPheno.spc.BLSSM_HEscan_'+str(i)
# Creating the new LesHouches file, and runing Spheno.
lhs.new_file(new_lhs_name)
param_card_path = SPhenoBLSSM.run(new_lhs_name,out_spheno_name)
# Reading spheno output
if not(param_card_path==None):
points = points + 1
index.append(i)
with open('number_of_points.txt','w+') as f:
f.write(f'{points} \n')
for i in index:
f.write(f'{i} \n')
| a3d38fb418350e9d20a775fe85089b16fcd52aa5 | [
"Markdown",
"Python",
"Shell"
] | 15 | Shell | mjadiaz/BL-SSM | 09cd0168dfe26da443525b0a4f6aa84e0491db37 | 1d45295bf3bde1e6896c9aafcfa33bdbc73f99b0 | |
refs/heads/master | <file_sep>import React from 'react'
import { withTracker } from 'meteor/react-meteor-data'
import { Store } from '/ui/Store.js'
const Msg = (props) => {
const { msg, type } = props.notification
return <div className={type}>{msg}</div>
}
export const Notification =
withTracker(() => {
return { notification: Store.notification.get() }
})(Msg)
<file_sep>import React from 'react'
import { Notification } from '/ui/Notification.jsx'
import { LogIn } from '/ui/LogIn.jsx'
import { Admin } from '/ui/Admin.jsx'
import { Guest } from '/ui/Guest.jsx'
export const App = () => (
<div>
<Notification/>
<h1>WebBlind - Audio Broadcasting with Meteor.js</h1>
<LogIn/>
<Admin/>
<Guest/>
</div>
)
<file_sep>import React, { useState } from 'react'
import styled from 'styled-components'
import { withTracker } from 'meteor/react-meteor-data'
import { AudioStreams } from '/collections/AudioStreams.js'
const GuestInner = (props) => {
const { audioStream, userId } = props
if (userId || !audioStream) return <></>
return <Styles>
<audio controls autoplay><source src={audioStream.file}/></audio>
</Styles>
}
export const Guest =
withTracker(() => {
Meteor.subscribe('AudioStreams.all')
const audioStream = AudioStreams.findOne() // TODO timing related stuff
console.log(audioStream)
const userId = Meteor.userId()
return ({ audioStream, userId })
})(GuestInner)
const Styles = styled.div`
`<file_sep>import React, { useState } from 'react'
import styled from 'styled-components'
import { withTracker } from 'meteor/react-meteor-data'
import { loadingWrapper, warn } from '/ui/Helpers.js'
import { AudioStreams } from '/collections/AudioStreams.js'
const AdminInner = (props) => {
const { audioStreams, isLoading, userId } = props
const add = () => event => {
event.preventDefault()
if (!file || !startDate || !startTime) {
return warn('Please provide a file a start date and start time')
}
AudioStreams.insert({
startDate,
startTime,
file,
})
}
const remove = audioStream => event => AudioStreams.remove(audioStream._id)
const [file, setFile] = useState('')
const [startDate, setStartDate] = useState('')
const [startTime, setStartTime] = useState('')
const setValue = setter => event => setter(event.target.value.trim())
if (!userId) return <></>
return (
<Styles>
{loadingWrapper(isLoading, (() => <>
<form>
{/* <label>File: <input type="file" accept=".mp3" onChange={inputFile}/></label> */}
<label>File: <input type="text" onChange={setValue(setFile)}/></label>
<label>Start date: <input type="date" name="startDate" onChange={setValue(setStartDate)}/></label>
<label>Start time: <input type="time" name="startTime" onChange={setValue(setStartTime)}/></label>
<button onClick={add()}>Add</button>
</form>
<table>
<thead>
<tr>
<th>File</th>
<th>Start time</th>
<th></th>
</tr>
</thead>
<tbody>
{audioStreams.map((audioStream, i) =>
<tr key={i}>
<td>{audioStream.file}</td>
<td>{audioStream.startTime}</td>
<td>
<button onClick={remove(audioStream)}>Remove</button>
<audio controls><source src={audioStream.file}/></audio>
</td>
</tr>
)}
</tbody>
</table>
</>))}
</Styles>
)
}
export const Admin =
withTracker(() => {
const handle = Meteor.subscribe('AudioStreams.all')
const userId = Meteor.userId()
return ({ audioStreams: AudioStreams.find().fetch(), isLoading: !handle.ready(), userId })
})(AdminInner)
const Styles = styled.div`
form {
display: flex;
flex-direction: column;
width: 30%;
margin-bottom: 1rem;
}
table {
border-spacing: 0;
border: 1px solid grey;
tr {
:last-child {
td {
border-bottom: 0;
}
}
}
th,
td {
margin: 0;
padding: 0.5rem;
border-bottom: 1px solid grey;
border-right: 1px solid grey;
word-break: keep-all;
:last-child {
border-right: 0;
}
}
}
`<file_sep>import { Meteor } from 'meteor/meteor'
import { AudioStreams } from '/collections/AudioStreams.js'
const USERS = [
{ username: 'Test', email: '<EMAIL>', password: '<PASSWORD>', verified: true },
]
const AUDIO_STREAMS = [
{ file: 'https://www.computerhope.com/jargon/m/example.mp3', startDate: '2020-01-14', startTime: '01:01' },
]
export const loadInitialData = () => {
Meteor.users.remove({})
AudioStreams.remove({})
if (!Meteor.users.find().count()) {
USERS.forEach(user => Accounts.createUser(user))
AUDIO_STREAMS.forEach(stream => AudioStreams.insert(stream))
}
}<file_sep>import { Meteor } from 'meteor/meteor';
import { loadInitialData } from '/server/initial-data';
Meteor.startup(() => {
// DON'T CHANGE THE NEXT LINE
loadInitialData();
// YOU CAN DO WHATEVER YOU WANT HERE
}); | bae37d8c30e569706405bd5507ca9d7217af5924 | [
"JavaScript"
] | 6 | JavaScript | softwarerero/webblind-exam | 586f0c2613f01fff3e210df0222e0e8272717f25 | 25443318409120510721293a3ba845feff9bc7fa | |
refs/heads/master | <file_sep>#!/usr/bin/python
import re, os, sys, glob, fnmatch
#doubleHome = os.path.dirname(os.path.dirname(os.path.dirname(os.environ["ROOTFS"])))
matches = []
for root, dirnames, filenames in os.walk('.'):
for filename in fnmatch.filter(filenames, '*.pri'):
fullName = os.path.join(root, filename)
found = False
with open(fullName) as fl:
if 'nano_441' in fl.read():
matches.append(fullName)
print fullName
else:
print ' skip ', fullName
for filename in matches:
bkName = filename+'.bak'
os.system('mv '+filename+' '+bkName)
with open(bkName) as inF:
with open(filename, 'w') as outF:
lines = inF.readlines()
for line in lines:
line = line.replace("/home/manu/cross/nano_441", "$ROOTFS")
outF.write(line)
<file_sep># delete config.cache
# sudo apt install '.*libxcb.*' libxrender-dev libxi-dev libfontconfig1-dev libudev-dev libxkbcommon-dev
# apt download libgles2-mesa-dev libegl1-mesa-dev
# mkdir GLES EGL
# cd GLES; ar x ../libgles2*.deb; tar xf data.tar.xz
# cd EGL; ar x ../libegl1*.deb; tar xf data.tar.xz
# need to copy KHR as well
# copy to /usr/include
# sudo usermod -a -G input manu //reboot takes effect logout?
# then
# add .bashrc
# *** modify qtbase/mkspecs/devices/linux-jetson-tx1-g++/qmake.conf
# QMAKE_INCDIR_POST /usr/include becomes to /usr/include/drm since pkg-config disabled automatically and need drm for egldevice
# change to eglfs_kms_egldevice
# see ../qmake.conf
# ***
#QT_PATH=/usr/local/qt5
#export PATH=${QT_PATH}/bin:$PATH
#export LD_LIBRARY_PATH=${QT_PATH}/lib:${LD_LIBRARY_PATH}
#nano works
./configure -shared -c++std c++14 -opensource -release -recheck-all --confirm-license -device linux-jetson-tx1-g++ -device-option CROSS_COMPILE=aarch64-linux-gnu- -prefix /usr/local/qt5 -extprefix /usr/local/qt5 -hostprefix /usr/local/qt5 -skip webview -skip qtwebengine -opengl es2
#nano fail
./configure -shared -c++std c++14 -opensource -release -recheck-all --confirm-license -device linux-jetson-tx1-g++ -device-option CROSS_COMPILE=aarch64-linux-gnu- -nomake examples -nomake tests -prefix /usr/lib/qt5.14.1 -skip webview -skip qtwebengine -opengl es2
#x86
./configure -shared -c++std c++14 -opensource -release -recheck-all --confirm-license -device linux-jetson-tx1-g++ -device-option CROSS_COMPILE=/home/manu/bin/gcc-linaro-6.5.0-2018.12-x86_64_aarch64-linux-gnu/bin/aarch64-linux-gnu- -sysroot /home/manu/jetson -nomake examples -nomake tests -prefix /usr/local/qt5 -extprefix /home/manu/Downloads/qt-everywhere-src-5.14.1/NanoR32.3.1/qt5 -hostprefix /home/manu/Downloads/qt-everywhere-src-5.14.1/NanoR32.3.1/qt5-host -skip webview -skip qtwebengine -opengl es2
#not this
./configure -shared -c++std c++14 \
-opensource -release --confirm-license -no-pkg-config \
-device linux-jetson-tx1-g++ \
-device-option CROSS_COMPILE=/home/manu/bin/gcc-linaro-6.5.0-2018.12-x86_64_aarch64-linux-gnu/bin/aarch64-linux-gnu- \
-sysroot /home/manu/jetson \
-nomake examples -nomake tests \
-prefix /usr/local/qt5 \
-extprefix /home/manu/Downloads/qt-everywhere-src-5.14.1/NanoR32.3.1/qt5 \
-hostprefix /home/manu/Downloads/qt-everywhere-src-5.14.1/NanoR32.3.1/qt5-host \
-skip webview -skip qtwebengine -opengl es2
./configure -shared -c++std c++14 \
-opensource -release --confirm-license -no-pkg-config \
-device linux-jetson-tx1-g++ \
-device-option CROSS_COMPILE=/home/manu/bin/gcc-linaro-6.5.0-2018.12-x86_64_aarch64-linux-gnu/bin/aarch64-linux-gnu- \
-sysroot /home/manu/Downloads/nano-rootfs \
-nomake examples -nomake tests \
-prefix /usr/local/qt5 \
-extprefix /home/manu/Downloads/qt-everywhere-src-5.14.1/JetsonNano/qt5 \
-hostprefix /home/manu/Downloads/qt-everywhere-src-5.14.1/JetsonNano/qt5-host \
-skip webview -skip qtwebengine -opengl es2
./configure -shared -c++std c++14 \
-opensource -release --confirm-license -no-pkg-config \
-device linux-jetson-tx1-g++ \
-device-option CROSS_COMPILE=/home/manu/bin/gcc-linaro-5.5.0-2017.10-x86_64_aarch64-linux-gnu/bin/aarch64-linux-gnu- \
-sysroot /home/manu/Downloads/nano-rootfs \
-nomake examples -nomake tests \
-prefix /usr/local/qt5 \
-extprefix /home/manu/Downloads/qt-everywhere-src-5.14.1/JetsonNano/qt5 \
-hostprefix /home/manu/Downloads/qt-everywhere-src-5.14.1/JetsonNano/qt5-host \
-skip webview -skip qtwebengine -opengl es2
<file_sep>$myID = 'TREE'
$myPW = '<PASSWORD>'
$myLink = "dinbendon.net"
require 'watir-webdriver'
#give options default value
$options = {'debug' => 0}
#
# output message if message level is no less than current setting
#
def dbg(msg, msgLevel = 1)
if $options['debug'] >= msgLevel then
puts "#{msg}"
end
end
def fire(link)
bw = Watir::Browser.new :chrome
bw.goto link
bw.body.div(:id => 'main').table.table(:class => 'lists').tbody.wait_until_present
tbody1 = bw.body.div(:id => 'main').table.table(:class => 'lists').tbody
if NIL == tbody1 then
puts "No tbody found"
return
end
tbody1.text_field(:name => 'username').set $myID
tbody1.text_field(:name => 'password').set $myPW
#puts "#{tbody1.trs[2].inspect}, #{tbody1.trs[2].text}"
#puts "#{tbody1.trs[2].text}".match('\d+')
add1 = "#{tbody1.trs[2].text}".match(/(\d+)([^\d]*)(\d+)/)[1]
add2 = "#{tbody1.trs[2].text}".match(/(\d+)([^\d]*)(\d+)/)[3]
rlt = add1.to_i + add2.to_i
dbg "#{add1} + #{add2} = #{rlt}"
input = tbody1.text_field(:name => 'result')
input.hover
input.set rlt
dbg "#{tbody1.trs[4].inspect}"
dbg "#{tbody1.trs[4].td.inspect}"
dbg "#{tbody1.trs[4].tds[1].input.inspect}"
#this fails tbody1.trs[4].text_field(:name => 'submit').click
tbody1.trs[4].tds[1].input.click
end
###
# main
fire $myLink
<file_sep>#!/bin/bash
my_array=(abc def ghi)
for ((i=0; i<${#my_array[@]}; i++ )); do echo ${my_array[$i]}; done
echo ${my_array[*]}
for ((i=0; i<${#my_array[@]}; i++ )); do echo ${my_array[i]}; done
<file_sep>#!/bin/bash
# run after
# docker exec -it mj /bin/bash
tasks=(
"check_space"
"clean_yyyymm"
"copy_to_release"
"edge_be"
"edge_branch"
"edge_fe"
"edge_pipe"
"edge_v20"
"q8_buildroot"
"q8_branch"
"q8_client"
"q8_pipe"
"q8_server"
"q8_webui"
"q8_v02"
"release_to_version"
)
#replaced by edge_pipe "edge_sys"
#replaced by edge_pipe "edge_system"
#edge_sys and edge_system deprecate
for ((i=0; i<${#tasks[@]}; i++)); do
echo Getting task ${tasks[i]}
java -jar jenkins-cli.jar -auth manu:manu -s http://localhost:8080 get-job ${tasks[i]} > ${tasks[i]}.xml
done
<file_sep>#!/bin/bash
OK=0
CANCEL=1
ESC=255
BACKTITLE="Cody Blog - Example"
Menu(){
while :
do {
Selection=$(dialog --title "CodyBlog - Menu" --clear \
--backtitle "$BACKTITLE" \
--cancel-label "Exit" \
--menu "Choose one" 12 45 5 \
1 "Test 01" \
2 "Test 02" \
2>&1 > /dev/tty)
result=$?
if [ $result -eq $OK ]; then
Select $Selection
elif [ $result -eq $CANCEL ] || [ $result -eq $ESC ]; then
Exit
fi
} done
}
Select(){
Choice=$1
case $Choice in
1) # Option 1
Option1
;;
2) # Option 2
Option2
;;
esac
}
Option1(){
while :
do {
exec 3>&1
Input=$(dialog --title "$Title" --clear \
--backtitle "$BACKTITLE" \
--form "Please input:" 10 70 5 \
"Input1:" 1 2 "" 1 14 48 0 \
"Input2:" 2 2 "" 2 14 48 0 \
2>&1 1>&3)
result=$?
exec 3>&-
IFS=$'\n'
Values=($Input)
unset IFS
if [ $result -eq $CANCEL ] || [ $result -eq $ESC ]; then
break
elif [ $result -eq $OK ]; then
MsgBox "${Values[0]}" "${Values[1]}"
if [ $result -eq $OK ]; then
break
fi
fi
} done
}
Option2(){
while :
do {
Number=$(dialog --title 'Option 2' --clear \
--backtitle "$BACKTITLE" \
--radiolist 'Select Item:' 15 70 2 \
1 Radiolist1 off \
2 Radiolist2 on \
2>&1 > /dev/tty)
result=$?
if [ $result -eq $CANCEL ] || [ $result -eq $ESC ]; then
break
elif [ $result -eq $OK ]; then
MsgBox "$Number"
break
fi
} done
}
MsgBox(){
Input1=$1
Input2=$2
Msg="$Input1\n"
Msg="${Msg}$Input2"
dialog --title "MsgBox" --clear \
--backtitle "$BACKTITLE" \
--yesno "$Msg" 10 70
result=$?
}
Exit(){
clear
echo "Program Terminated."
exit
}
Menu
<file_sep># Download base image manu-jenkins:0000
# docker build -t manu-jenkins:0100 .
# RUN:
# docker run -d --name mj --user root -p 8080:8080 -p 50000:50000 -v /var/run/docker.sock:/var/run/docker.sock -v <host_jenkins_home>:/var/jenkins_home manu-jenkins:0100
# Check password: in /var/jenkins_home/secrets/initialAdminPassword
# docker exec -it mj /bin/bash
# NOTE:
# jenkins shell use sh (dash), at top of script use "#!/bin/bash" without any blank to make .bashrc working
# 00 is major 00 is minor
# 01 is major 00 is minor
# must have USER root to avoid apt-key or something fail
#
# Suggested modules:
# 1. Copy Artifact
# 2. Pipeline Utility Steps
FROM manu-jenkins:0000
LABEL maintainer="<EMAIL>"
LABEL version="01.00"
LABEL description="jenkins for Q8Vista"
USER root
ENV VER=1.14.15
ENV APP="make autoconf automake autopoint bison flex gperf libtool libtool-bin libtool lzip p7zip-full intltool-debian intltool libgtk2.0-dev python ruby npm wget cpio rsync bc gcc-mingw-w64 g++-mingw-w64 libssl-dev libelf-dev gtk-doc-tools"
RUN apt-get update && apt-get -y install $APP
RUN wget -c https://dl.google.com/go/go${VER}.linux-amd64.tar.gz -O - | tar -xz -C /usr/local && echo "export GOROOT=/usr/local/go" >>~/.bashrc && echo "export PATH=\${PATH}:\${GOROOT}/bin" >>~/.bashrc
<file_sep>#!/bin/sh
#
# In : $1- message
#
function usage()
{
test ! -z "$1" && echo "Error: $1"
echo " Usage: $0 FILE"
echo " To remove comments in FILE"
exit 0
}
#
# main
#
# check parameter count & file existence
#
test $# != 1 && usage "Incorrect number of parameter !!!"
test ! -f $1 && usage "$1 doesn't exist !!!"
# remove line comment
#
cat $1 | sed 's/\/\/.*$//g' >$1.line
# remove block comment in a line
#
cat $1.line | sed 's/\/\*.*\*\///g' >$1.block1
# remove block comment in multiple lines
#
cat $1.block1 | sed 's/\/\*\(.*^\)\+.*\*\///g' >$1.block2
<file_sep>#!/bin/bash
#if parameter is not set, use default
parameter-default() {
local var1=1
local var2=2
echo "var1=$var1, var2=$var2"
echo " var1 defined, \${var1-\$var2} outputs \$var1 (${var1-$var2})"
echo " var3 not defined, \${var3-\$var2} outputs \$var2 (${var3-$var2})"
echo
var1=
echo "var1="
echo " var1 defined, \${var1-\$var2} outputs \$var1 (${var1-$var2})"
echo " var1 declared but set as null, \${var1:-\$var2} outputs \$var2 (${var1:-$var2})"
echo
}
#if parameter is not set, set it to default
parameter-set-default() {
echo "varA is not declared"
echo " \${varA=abc} sets var as (${varA=abc})"
echo "${varA:=xyz}" >/dev/null
echo " this time \${varA=xyz} leaves varA remain (${varA})"
echo
local varB=
echo " varB declared but is set as null, \${varB=abc} leaves varB as (${varB=abc})"
echo " varB declared but is set as null, \${varB:=abc} sets varB as (${varB:=abc})"
echo
}
parameter-default
parameter-set-default
aa='echo $MODEL'
MODEL=4
echo `eval ${aa}`
<file_sep># use ${ROOTFS} of jetson sample_rootfs (copy from nano success)
# delete config.cache
# qtbase/mkspecs/devices/linux-jetson-tx1-g++/qmake.conf
# modify eglfs_x11 as eglfs_kms_egldevice
# set ROOTFS
# set PATH to gcc-linaro-7.3.1-2018.05-x86_64_aarch64-linux-gnu (jetson developer)
#test this
./configure -shared -c++std c++14 -opensource -release -recheck-all --confirm-license\
-device linux-jetson-tx1-g++ -device-option CROSS_COMPILE=aarch64-linux-gnu-\
-sysroot ${ROOTFS} -nomake tests -prefix /usr/local/qt5 -extprefix /usr/local/qt5 -hostprefix /usr/local/qt5 -skip webview -skip qtwebengine -opengl es2
<file_sep>#!/bin/bash
PS3="Choose: "
select i in a336 c039 quit
do
[ "$i" = "quit" ] && exit 0
echo "You chose $i"
break #otherwise user has to choose again and again
done
===================================
the list of select can be omit and it will default to "$@", i.e., the list of quoted command-line arguments.
===================================
print 'Select your terminal type:'
PS3='terminal? '
select term in \
'Givalt VT100' \
'Tsoris VT220' \
'Shande VT320' \
'Vey VT520'
do
case $REPLY in
1 ) TERM=gl35a ;;
2 ) TERM=t2000 ;;
3 ) TERM=s531 ;;
4 ) TERM=vt99 ;;
* ) print 'invalid.' ;;
esac
if [[ -n $term ]]; then
print TERM is $TERM
break
fi
done
<file_sep>#!/bin/bash
D4_MAX_PACKET=3045
D4_P_NAME="VP2730 UVC Device"
VID=1367
PID=10244
VENDOR_NAME="ATEN International Co.,Ltd"
CMD_PREFIX=
P_NAME=
MAX_PACKET=
#
# Usage:
# gouvc [-p PRODUCT_NAME] [-x MAX_PACKET]
#
#
usage() {
echo "Usage: $0 [-p PRODUCT_NAME] [-x MAX_PACKET]"
echo " PRODUCT_NAME: product name, default to ${D4_P_NAME}"
echo " MAX_PACKET: max packet, yuv defaults to ${D4_MAX_PACKET}"
}
#
# Update g_xxx variable
#
parse_param() {
while getopts ":p:x:" opt; do
case $opt in
p) P_NAME=$OPTARG;;
x) MAX_PACKET=$OPTARG;;
\?) usage
exit;;
esac
done
OPTIND=1
test -z "${P_NAME}" && P_NAME=${D4_P_NAME}
test -z ${MAX_PACKET} && MAX_PACKET=${D4_MAX_PACKET}
test 0 -eq `id|grep root|wc -l` && CMD_PREFIX="sudo "
#echo "command=$CMD_PREFIX, $P_NAME, $MAX_PACKET"
}
#
# Remove driver
#
rmdriver() {
${CMD_PREFIX}rmmod g_webcam >/dev/null 2>&1
s{CMD_PREFIX}rmmod usb_f_uvc >/dev/null 2>&1
}
#
# Remove driver
#
lddriver(){
${CMD_PREFIX}modprobe g_webcam idVendor=${VID} idProduct=${PID}\
iSerialNumber="28032" iManufacturer=\"${VENDOR_NAME}\"\
iProduct=\"${P_NAME}\" streaming_maxpacket=${MAX_PACKET}
}
########
# main
########
parse_param "$@"
rmdriver
lddriver
sleep 1
./uvc-gadget -d -u/dev/video0
<file_sep>#!/bin/sh
#
# To remove modified cvs files (local)
#
# Note: make sure modified source are committed separately
#
#cvs -n update | grep "^M" | awk '{print $2}' | xargs rm
cvs -n update | grep "^?" | awk '{print $2}'
<file_sep>#usage: ini.rb INI_FILE
#give options default value
$options = {'ini_dbg' => 0, 'print' => false}
#
# output message if message level is no less than current setting
#
def iniDbg(msg, msgLevel = 1)
if $options['ini_dbg'] >= msgLevel then
puts "#{msg}"
end
end
# Get array with given INI file
# In : filaName
# Ret : hash, name after session, of hash
def iniGet(fileName)
hashOfHash = {} #SESSION => hashOfSession
curHash = {} #KEY => value
curSess = 'Null'
File.foreach(fileName) do |line|
line = line.chomp.strip #remove leading and trailing spaces and newline
next if line =~ /^;/ #skip comment line
next if line.length == 0 #skip empty line
if line =~ /^\[(.+)\]/ then #session found
tmpHash = curHash.clone #copy hash to insert in hash since curHash will be modified
if !curHash.empty? then
cmd = "hashOfHash[\'" + "#{curSess}'\] = tmpHash"
iniDbg("#{cmd}", 2)
eval(cmd)
elsif curSess != 'Null' then
cmd = "hashOfHash[\'" + "#{curSess}'\] = tmpHash"
iniDbg("#{cmd}", 2)
eval(cmd)
end
curHash.clear
iniDbg("found session #{curSess}")
next if curSess = $1 #this must be a true assignment
end
token = line.split('=', 2) #cut to two pieces at most
iniDbg(" \(key, value\) = \(#{token[0]}, #{token[1]}\)")
cmd = "curHash\['" + "#{token[0]}'\] = " + "'#{token[1]}'"
iniDbg("#{cmd}", 2)
eval(cmd)
end
#the last session
cmd = "hashOfHash[\'" + "#{curSess}'\] = curHash"
iniDbg("#{cmd}", 2)
eval(cmd)
return hashOfHash
end
###
# main
if $0 == 'ini.rb' then
require 'optparse'
OptionParser.new do |opts|
opts.banner = "Usage: ruby ini.rb [-f INI_FILE] [-d LEVEL] [-p]
LEVEL defaults to 0, which means debug off
INI_FILE ini file for parsing
-p to print session and content, defaults to off"
opts.on('-d', '--debug level', 'debug level') { |level|
$options['ini_dbg'] = level.to_i
}
opts.on('-f', '--file INI_file', 'INI file for parsing') { |file|
$options['file'] = file
}
opts.on('-p', '--print', 'print sessions and content') {
$options['print'] = true
}
opts.on('-h', '--help', 'Display usage') {
puts "#{opts.banner}"
exit
}
end.parse(ARGV)
if !$options.has_key?('file') then
puts "No INI file provided"
exit
else
iniDbg("Processing #{$options['file']} ...")
end
hh = iniGet($options['file'])
if $options['print'] then
hh.each_pair do |session, hash|
puts "----- session \"#{session}\" -----"
hash.each_pair do |key, value|
puts " #{key} = #{value}"
end
end
end
end
<file_sep>#!/bin/bash
# dump given size from memory to current dir
# $1 dump size, must >= 4096, and round down to 4K
# can be number of xxxK, xxxM, xxxG
#
# dump2file FILE or
# if FILE present, it must contain "USB=[PATH]/[FNAME]"
# or "HD=[PATH]/[FNAME]" or "NFS=[PATH]/[FNAME]"
#
# dump2file SIZE or
# dump2file SIZE INPUT_FILE or
# dump2file SIZE INPUT_FILE OUTPUT_FILE
#
# INUPT_FILE defaults to /dev/zero
# OUTPUT_FILE defaults to dmp2file_SIZE
file_path=`dirname $0`
. $file_path/func
#
#parameter check
#
test -z $1 && echo Error paramter && exit
test -f $1 && doFile $1 && exit
#get size
fsize=`expr 1024 \* 1024 \* 1024`
usrSize=$1
case $usrSize in
*G|*g)
usrSize=`echo $usrSize |sed -e s/[Gg]//`
fsize=`expr $usrSize \* $SIZE_1G`
;;
*M|*m)
usrSize=`echo $usrSize |sed -e s/[Mm]//`
fsize=`expr $usrSize \* $SIZE_1M`
;;
*K|*k)
usrSize=`echo $usrSize |sed -e s/[Kk]//`
fsize=`expr $usrSize \* $SIZE_1K`
;;
*)
fsize=$usrSize
;;
esac
#get file name
inFile=/dev/zero
test ! -z $2 && inFile=$2
outFile=$3
checkSize $fsize
test -z $outFile && outFile=dmp2file_$DISPLAY_SIZE
test ! -c $outFile && rm -f $outFile
echo "Dump from $inFile to $outFile, size=$DUMP_SIZE"
doDump $inFile $outFile $BLOCK_SIZE $COUNT
<file_sep>#!/bin/sh
# check usage
TGTDIR=
#relative to kernel tree
SUBDIR=
GIT_SRC=
TGTTAG=master
IS_HTTP=
#
# Usage:
# dirdiff -s SRC_DIR [-t TGT_DIR] [-c]
#
# show difference of file name between SRC_DIR and TGT_DIR
# those missing in TGT_DIR can be copied from SRC_DIR if -c specified
#
usage() {
echo "Usage: $0 -s GIT_SRC [-d DIR_NAME] [-p RELATIVE_PATH] [-t TAG]"
echo " ex. $0 -s https://github.com/manuTW/linux -p drivers/media -t v3.12"
echo " -s : use file, git://, or https:// protocal to get GIT_SRC"
echo " -d : checkout directory name"
echo " -p : relative path without \"/\" from GIT_SRC to checkout, default as all GIT_SRC"
echo " -t : default to master if not provided"
exit
}
#parse and do checkout
parse_param() {
while getopts ":s:p:t:d" opt; do
case $opt in
s) GIT_SRC=$OPTARG;;
d) TGTDIR=$OPTARG;;
p) SUBDIR=$OPTARG;;
t) TGTTAG=$OPTARG;;
\?) usage;;
esac
done
OPTIND=1
test -z "${GIT_SRC}" && echo Missing GIT_SRC && usage
#remove tailing ".git" if any
test -z "${TGTDIR}" && TGTDIR=`basename ${GIT_SRC} | sed s/\.git$//`
IS_HTTP=`echo ${GIT_SRC}|grep -i http`
TGTDIR=${TGTDIR}-`date +"%m%d_%H%M%S"`
if [ -z "${SUBDIR}" ]; then
echo Checkout ${GIT_SRC} to ${TGTDIR}, tag=${TGTTAG}
else
echo Checkout ${SUBDIR} of ${GIT_SRC} to ${TGTDIR}, tag=${TGTTAG}
fi
}
parse_param $@
mkdir -p ${TGTDIR}; cd ${TGTDIR}
git init
git remote add -f origin ${GIT_SRC}
git fetch origin
git config core.sparseCheckout true
test -n "${SUBDIR}" && echo "${SUBDIR}" > .git/info/sparse-checkout
if [ ${TGTTAG} = "master" ]; then
git checkout master
else
git checkout -b br_${TGTTAG} ${TGTTAG}
fi
<file_sep>#!/bin/bash
# Usage: new_install manuchen
IP_KVM=172.16.58.3
IP_NAS=192.168.127.12
IP_PC=192.168.127.12
IP_SERVER=172.16.31.10
PATH_GITHUB=~/work/gh
PATH_NAS=~/nas
PATH_SHELL=${PATH_GITHUB}/shell
URL_GIT_SHELL="https://github.com/twManu/shell.git"
APPLIST="gitk vim cifs-utils minicom v4l-utils terminator fcitx-libs-dev fcitx-table fcitx-tools gcin gcin-tables"
#
# in : S1 - user name to set permision with
#
get_param()
{
if [ -z "$1" ]; then
G_USER=`whoami`
else
G_USER=$1
fi
X64_CPU=`uname -m`
MY_UID=`id $G_USER | cut -d"=" -f2 | cut -d"(" -f1`
test -z "$MY_UID" && exit
echo G_USER = $G_USER, X64_CPU = $X64_CPU, MY_UID = $MY_UID
}
#
# get answer among (y/n/s)
# in : $1 - string to highligh for user
# out : g_ANS = 'n' or 's' or default = 'y'
#
get_answer()
{
MESSAGE=$1
echo -en "\\033[0;33m"
echo -en "$MESSAGE (Yes/No/Skip): "
echo -en "\\033[0;39m"
read ans
if [ $ans == 'N' -o $ans == 'n' ]; then
g_ANS='n'
elif [ $ans == 'S' -o $ans == 's' ]; then
g_ANS='s'
else
g_ANS='y'
fi
}
#
# setting .bashrc
#
do_bashrc()
{
result=`grep smbnas ~/.bashrc`
if [ -n "${result}" ]; then
get_answer "The bashrc might be updated, continue to process ?"
if [ $g_ANS == 'n' ]; then
exit 0
elif [ $g_ANS == 's' ]; then
return
fi
fi
mkdir -p ${PATH_NAS} ${PATH_GITHUB}
echo "alias smbnas='sudo mount.cifs //${IP_NAS}/share/git ~/nas -o uid=\$(id -u),gid=\$(id -g),username=manu,vers=1.0'" >>~/.bashrc
echo "export PATH=${PATH_SHELL}:\${PATH}" >>~/.bashrc
test ! -d ${PATH_SHELL} && cd ${PATH_GITHUB}; git clone ${URL_GIT_SHELL}
}
#
# install applications
#
do_app_install()
{
APPLIST="gitk vim cifs-utils minicom v4l-utils terminator virtualenv virtualenvwrapper python3.8 python3.8-dev python3.8-venv apt-utils libhdf5-dev libffi-dev libxml2-dev libxslt1-dev"
#for dayi-ibus
APPLIST="${APPLIST} uuid-runtime"
test $X64_CPU = "x86_64" && APPLIST="$APPLIST ia32-libs lib32ncurses5-dev lib32z1-dev lib32readline-gplv2-dev"
get_answer "Proceed to install $APPLIST ?"
if [ $g_ANS == 'n' ]; then
exit 0
elif [ $g_ANS == 's' ]; then
return
fi
sudo apt update
sudo apt install -y $APPLIST
sudo snap install barrier
}
#
# configure samba
# in : $1 - username
#
do_smb()
{
SYSSMBCFG=/etc/samba/smb.conf
SMBCFG=./tmp.conf
if grep $G_USER $SYSSMBCFG; then
get_answer "The $G_USER is present in smb.conf, continue to process ?"
if [ $g_ANS == 'n' ]; then
exit 0
elif [ $g_ANS == 's' ]; then
return
fi
fi
if [ -f $SYSSMBCFG ]; then
cp $SYSSMBCFG $SMBCFG
echo "" >> $SMBCFG
echo "[$G_USER]" >> $SMBCFG
echo " path = /home/$G_USER/" >> $SMBCFG
echo " browseable = yes" >> $SMBCFG
echo " read only = no" >> $SMBCFG
echo " guest ok = no" >> $SMBCFG
echo " create mask = 0664" >> $SMBCFG
echo " directory mask = 0755" >> $SMBCFG
echo " force user = $G_USER" >> $SMBCFG
echo " force group = $G_USER" >> $SMBCFG
sudo mv $SMBCFG $SYSSMBCFG
fi
if [ ! -z `which smbpasswd` ]; then
sudo smbpasswd -L -a $G_USER
sudo smbpasswd -L -e $G_USER
fi
}
#NOTE:
#1.the mount.cifs uid=<uid#>, the user name becames unavailable after 11.10
#2.the ia32-libs fails x86 installation
get_param $1
do_app_install
do_bashrc
<file_sep>function basher() {
if [[ $1 = 'run' ]]
then
shift
/usr/bin/docker run -e \
HIST_FILE=/root/.bash_history \
-v $HOME/.bash_history:/root/.bash_history \
"$@"
else
/usr/bin/docker "$@"
fi
}
alias docker=basher<file_sep>#!/bin/bash
g_SRC_DIR=
g_TGT_DIR=.
g_ACT_COPY=0
#
# Usage:
# dirdiff -s SRC_DIR [-t TGT_DIR] [-c]
#
# show difference of file name between SRC_DIR and TGT_DIR
# those missing in TGT_DIR can be copied from SRC_DIR if -c specified
#
usage() {
echo "USage: dirdiff -s SRC_DIR [-t TGT_DIR] [-c]"
echo " SRC_DIR: source directory"
echo " TGT_DIR: target directory, default as current directory"
echo " -c: copy those TGT_DIR missing"
}
#
# Update g_xxx variable
#
parse_param() {
while getopts ":s:t:c" opt; do
case $opt in
s) g_SRC_DIR=$OPTARG;;
t) g_TGT_DIR=$OPTARG;;
c) g_ACT_COPY=1;;
\?) usage
exit;;
esac
done
OPTIND=1
}
#
# Check if g_xxx variable valid
# ask for confirmation if to alter something
#
check_param() {
test -z ${g_TGT_DIR} && $g_TGT_DIR=$CWD
test ! -d $g_SRC_DIR && echo "Error: '$g_SRC_DIR' is not a valid source directory" && exit
#echo source dir=$g_SRC_DIR, target dir=$g_TGT_DIR, do copy=$g_ACT_COPY
if [ 0 != $g_ACT_COPY ]; then
echo -n " copy missing files of $g_TGT_DIR from $g_SRC_DIR? (y/n) "
read ans
case $ans in
y*|Y*) ;;
*) echo " ...stop processing !!!"
exit;;
esac
else
echo " show different file in $g_SRC_DIR and $g_TGT_DIR..."
fi
}
#
# show those source have but target doesn't
# $1 : source directory
# S2 : target directory
# $3 : prefix char (- or +)
process_1() {
local dir_list=
local count=0
local src
local tgt
local prefix
if [ $# -lt 3 ]; then
echo wrong argument number !!!
exit
else
src=$1; tgt=$2; prefix=$3
fi
for file in `ls -a $src`; do
((count++))
if [ -f $src/$file ]; then
test ! -f $tgt/$file && echo "${prefix}f $src/$file"
elif [ -d $src/$file ]; then
test ! -d $tgt/$file && echo "${prefix}d $src/$file"
if [ $count -gt 2 ]; then #skip . ..
if [ "$dir_list" == "" ]; then
dir_list=$file #avoid " abc", "abc" instead
else
dir_list="$dir_list $file"
fi
fi
else
test ! -e $tgt/$file && echo "${prefix} $src/$file"
fi
done
test ! -z "$dir_list" && echo "directory '${dir_list}' not compared !"
}
#
# show source has but target doesn't with + and - vice versa
# $1 : source directory
# S2 : target directory
#
process() {
local src
local tgt
test $# -lt 2 && echo wrong argument number !!! && exit
src=$1; tgt=$2
process_1 $src $tgt "-"
process_1 $tgt $src "+"
}
############
### main ###
############
parse_param $@
check_param
process $g_TGT_DIR $g_SRC_DIR
<file_sep>#!/bin/sh
CUSTROOT=/home/custom
find ${CUSTROOT} -maxdepth 2 | grep -e "-[0-9].*\.[0-9][0-9][1-9,a-c][0-9][0-9]$"
<file_sep># must build ubuntu2004:0000 in advance
# docker build -t ubuntu2004:0110 .
# 01 is major 00 is minor
# for go
#
FROM ubuntu2004:0000
LABEL maintainer="manuchen@home"
LABEL version="01.00"
LABEL description="base of go"
ENV VER=1.14.8
RUN wget -c https://dl.google.com/go/go${VER}.linux-amd64.tar.gz -O - | tar -xz -C /usr/local && echo "export GOROOT=/usr/local/go" >>~/.bashrc && echo "export PATH=\${PATH}:\${GOROOT}/bin" >>~/.bashrc
<file_sep>#!/bin/sh
#
# Taken scan.LOG as output of A180 debug message and format the stitistics
# in file tmp.log
#
# there are 37 channels in area of post code 95035
#
LOG_FILE=scan.LOG
TMP_FILE=tmp.log
STATISTIC_FILE=statistic.log
# extract critical information
#
cat $LOG_FILE | awk '/:ATSCTunerNodeGetSignalLocked|:ATSCTunerNodeGetSignalStrength|:ATSCTunerNodeGetSignalQuality|:SetATSCFrequency/ {print}' > $TMP_FILE
# format as
# freq (lock,strength,quality)
# for each iteration
cat $TMP_FILE | \
awk 'NR%4 == 1 { freq=$5 }; NR%4 == 2 { lock=$5 }; NR%4 == 3 { strength=$5 }; NR%4 == 0 \
{print freq, "("lock","strength","$5")"}' > $STATISTIC_FILE
# format as
# freq (lock,strength,quality) (lock,strength,quality)...
# for each distict frequency
FREQ_LIST=
cur_freq=
line=1
for i in `cat $STATISTIC_FILE`; do
if [ 1 == `expr $line % 2` ]; then # frequency
if [ ! "0${FREQ_COUNTs["$i"]}" -gt 0 ]; then
# remember each distinct frequency
FREQ_LIST="$FREQ_LIST $i"
FINAL_LISTs["$i"]=$i
let FREQ_COUNTs["$i"]++
fi
cur_freq=$i
else # (lock,strength,quality)
FINAL_LISTs["cur_freq"]="${FINAL_LISTs["cur_freq"]} $i"
fi
let line++
done
# print result
echo '============ statistics =======================================' >>$TMP_FILE
echo 'frequency (lock, strength, quality) (lock, strength, quality...' >>$TMP_FILE
echo '===============================================================' >>$TMP_FILE
for i in $FREQ_LIST; do
echo ${FINAL_LISTs["$i"]} >> $TMP_FILE
done
<file_sep>#!/bin/sh
#
# To touch the whole directory
#
# In : $1 - target directory
#
# In : $1 - given string to show
#
usage()
{
echo "$1"
echo "Usage: touchd.sh TARGET_DIR"
exit -1
}
test -z $1 && usage
test ! -d $1 && usage "\"$1\" does not exist !!!"
find $1 -exec touch '{}' \;
<file_sep>#!/bin/bash
hexdump -C ~/.bashrc
hexdump -x ~/.bashrc
<file_sep>export AM_DIR=~/bin/amlogic
export WK_DIR=$AM_DIR
export PATH=~/bin:$AM_DIR/arm-2010q1/bin:$PATH
export CROSS_COMPILE=arm-none-eabi-
export KSRC=$AM_DIR/arm-src-kernel-2011-02-21-11.30-git-9dababe0e2
export KOBJ=$KSRC
<file_sep># Download base image ubuntu 20.04
# docker build -t ubuntu2004:0000 .
# 00 is major 00 is minor, python3.8 by default
# g++
FROM --platform=linux/amd64 ubuntu:20.04
LABEL maintainer="<EMAIL>"
LABEL version="00.00"
LABEL description="base of ubuntu"
ENV TZ=Asia/Taipei
ENV APP="vim git curl build-essential g++ software-properties-common wget"
RUN ln -snf /usr/share/zoneinfo/$TZ /etc/localtime && echo $TZ > /etc/timezone
RUN apt-get update && apt-get install -y $APP
RUN echo EDITOR=vim >>~/.bashrc
<file_sep>#!/bin/bash
echo "udevadm info --query=all --name=usb"
echo "udevadm info --query=all --name=/dev/dri/card0 --attribute-walk"
echo "udevadm info --attribute-walk --path=$(udevadm info --query=path --name=/dev/dri/card0)"
echo "udevadm monitor"
echo "sudo udevadm control --reload"
echo "tail -f /var/log/usbdevice.log"
<file_sep>#!/bin/bash
sudo apt update
sudo apt download libgles2-mesa-dev libegl1-mesa-dev
mkdir GLES EGL
pushd GLES; ar x ../libgles2*.deb; tar xf data.tar.xz; sudo cp -a usr/include/GLES* /usr/include; popd
pushd EGL; ar x ../libegl1*.deb; tar xf data.tar.xz; sudo cp -a usr/include/EGL /usr/include; popd
cp -a /usr/src/nvidia/graphics_demos/include/KHR /usr/include
sudo apt install libglm-dev libinput-dev libudev-dev symlinks libxkbcommon-dev ".*libxcb.*" libxrender-dev libxi-dev libfontconfig1-dev
pushd /; sudo symlinks -c -r / ;tar cjf ~/nano441_root.tgz lib usr/include usr/lib usr/local/cuda*; popd
<file_sep>#!/bin/bash
# Usage: mountfdos FULL_PATH_IMAGE, /mnt/Data/KVM/fdos.img by default
MNT_DIR=/mnt/fdos
IMG=/mnt/Data/KVM/fdos.img
mkdir -p $MNT_DIR
test -n "$1" && IMG=$1
if [ -f $IMG ]; then
sudo mount -t msdos -o loop,offset=32256 $IMG $MNT_DIR
else
echo "Missing $IMG"
fi
<file_sep>#!/usr/bin/python
import glob, os
SCU="/sys/class/udc/"
UDC="dwc3.1.auto/"
ITEMS={
"a_alt_hnp_support": 1,
"current_speed": 1,
"is_a_peripheral": 1,
"maximum_speed": 1,
"srp": 0,
"uevent": 1,
"b_hnp_support": 1,
"is_otg": 0,
"power": 0,
"state": 1,
"b_hnp_enable": 1,
"function": 1,
"is_selfpowered": 0,
"soft_connect": 0,
"subsystem": 0
}
for ff in glob.glob(SCU+UDC+"*"):
basename = os.path.basename(ff)
if basename in ITEMS and ITEMS[basename]:
if os.path.islink(ff): continue
if os.path.isdir(ff): continue
print "*** checking", basename
os.system('cat '+ff)
<file_sep>#!/bin/bash
#Put the followings to [autoexec] of ~/.dosbox/san5.conf
# mount c ~/dos/san5
# mount d / -t cdrom
# c:
# san5.com
dosbox -conf ~/.dosbox/san5.conf
<file_sep># Usage:
# untar.sh -F|-R [-s SRC_FILE] -t TGT_DIR [-q]
#
#
# In : $1- message
#
function usage()
{
echo "Error: $1"
echo Usage:
echo "untar.sh -F|-R|-G [-s SRC_FILE] -t TGT_DIR [-q]"
echo " F : Fedora Core 3"
echo " R : Red Hat 9"
echo " G : Fedora Core 4"
echo " SRC_FILE: if omited, $RH9/$FC3 as default for R/F"
echo " TGT_DIR: target directory"
echo " q : quiet"
exit 0
}
#
# main
#
CUR_DIR=$PWD
RH9DIR=$CUR_DIR/RH9
RH9=rh9-root.tgz
FC3DIR=$CUR_DIR/FC3
FC3=fc3-root.tgz
FC4DIR=$CUR_DIR/FC4
FC4=fc4-root.tgz
D4_SRC_FILE=
SRC_FILE=
TGT_DIR=
QUIET= #not quiet by default
#
# 1. Check parameters
#
while getopts ":t:s:RFGBq" arg
do
case "$arg" in
t) TGT_DIR=$OPTARG;;
s) SRC_FILE=$OPTARG;;
F) D4_SRC_FILE=$FC3DIR/$FC3;;
R) D4_SRC_FILE=$RH9DIR/$RH9;;
G) D4_SRC_FILE=$FC4DIR/$FC4;;
q) QUIET=yes;;
esac
done
test -z $TGT_DIR && usage "No target directory assigned"
test ! -d $TGT_DIR && usage "Target directory $TGT_DIR doesn't exist"
test -z $D4_SRC_FILE && usage "Please use -F, -G or -R flag"
test -z $SRC_FILE && SRC_FILE=$D4_SRC_FILE
test ! -f $SRC_FILE && usage "File $SRC_FILE doesn't exist"
#
# 2. Determine source file type, what dir to create after install
#
case $D4_SRC_FILE in
*$FC3)
#tared "bin dev etc home initrd lib media misc opt root"
#tared "sbin selinux srv tftpboot usr var"
DIRS="boot mnt/usb proc sys tmp"
#not created lost+found
;;
*$FC4)
#tared "bin dev etc home lib media misc opt root"
#tared "sbin selinux srv tftpboot usr var"
DIRS="boot mnt/usb net proc sys tmp"
#not created lost+found
;;
*$RH9)
#tared "bin boot dev etc home initrd lib lost+found misc mnt"
#tared "opt proc root sbin tftpboot tmp usr var"
DIRS="mnt/usb"
;;
*) usage "Unknown system";;
esac
#
# guess format
#
if echo $SRC_FILE | grep -q -e "tgz$"; then
FLAGS="-xzf"
elif echo $SRC_FILE | grep -q -e "tar$"; then
FLAGS="-xf"
else
usage "Unknown source file format"
fi
if [ -z $QUIET ]; then
echo -n " To untar $FLAGS \"$TGT_DIR\" from $SRC_FILE ? (y/n): "
read ANSWER
if [[ x$ANSWER != xy && x$ANSWER != xY ]]; then exit 0; fi
fi
echo Processing...
pushd . >/dev/null 2>&1
cd $TGT_DIR;tar $FLAGS $SRC_FILE
if [ x"$DIRS" != x ]; then
echo Creating directories $DIRS ...
mkdir -p $DIRS
fi
popd >/dev/null 2>&1
<file_sep># Usage:
# tar.sh FC3|RH9|FC4 TGT_FILE SRC_DIR
#
#
# In : $1- message
#
function usage()
{
echo "Error: $1"
echo Usage:
echo "$0 FC3|RH9|FC4 TGT_FILE SRC_DIR"
exit 0
}
#
# check parameters
#
if [ "$#" != "3" ]; then usage "parameter count"; fi
if [ -d $3 ]; then SRC_DIR=$3; else usage "Directory doesn't exist"; fi
if [ ! -f $2 ]; then TGT_FILE=$2; else usage "File exist"; fi
case $1 in
FC3)
echo " **** you MUST boot with FC3 and then tar from / !!! ****"
DIR1="bin dev etc home initrd lib media misc opt root"
DIR2="sbin selinux srv tftpboot usr var"
# yet tared : boot lost+found mnt proc sys tmp
;;
FC4)
echo " **** you MUST boot with FC4 and then tar from / !!! ****"
DIR1="bin dev etc home lib media misc opt root"
DIR2="sbin selinux srv tftpboot usr var"
# yet tared : boot lost+found mnt net proc sys tmp
;;
RH9)
echo " **** you SHOULDN'T boot with RH9 and then tar from / !!! ****"
DIR1="bin boot dev etc home initrd lib lost+found misc mnt"
DIR2="opt proc root sbin tftpboot tmp usr var"
;;
*)
usage "Unknown system"
;;
esac
#
# guess target file
#
if echo $TGT_FILE | grep -q -v -e "^\/" ; then
TGT_FILE=`pwd`/$TGT_FILE
fi
#
# guess format
#
if echo $TGT_FILE | grep -q -e "tar$"; then
FLAGS="-c -p --same-owner -l -f"
ZIP=0
elif echo $TGT_FILE | grep -q -e "tgz$"; then
FLAGS="-c -p --same-owner -l -f -"
ZIP=1
else
usage "Unknown target file format"
fi
echo " To tar $FLAGS"
echo " $DIR1"
echo " $DIR2"
echo -n " from \"$SRC_DIR\" as $TGT_FILE ? (y/n): "
read ANSWER
if [[ x$ANSWER != xy && x$ANSWER != xY ]]; then exit 0; fi
echo Processing...
if [ -z "$ZIP" ]; then
cd $SRC_DIR;tar $FLAGS $TGT_FILE $DIR1 $DIR2
else
cd $SRC_DIR;tar $FLAGS $DIR1 $DIR2 | gzip -1 >$TGT_FILE
fi
<file_sep>#!/usr/bin/ruby
$myID = 'lessmoney'
$myPW = '<PASSWORD>'
$jkLink = "www.jkforum.net/forum.php"
$unLink = "twunbbs.com/forum.php"
$enLink = "www.eyny.com"
$st85Link = "85st.com/forum.php"
$fishLink = "fishgeter.com"
$allSites = {
'doJK' => "www.jkforum.net/forum.php"\
, 'doUn' => "twunbbs.com/forum.php"\
, 'doEyny' => "www.eyny.com"\
, 'do85ST' => "85st.com/forum.php"\
}
require 'watir-webdriver'
require 'optparse'
#give options default value
$options = {'debug' => 0}
#
# output message if message level is no less than current setting
#
def dbg(msg, msgLevel = 1)
if $options['debug'] >= msgLevel then
puts "#{msg}"
end
end
#
# set all options['xxx'] to given value
def setAllOptions(value)
$allSites.keys.each do |site|
cmd = "\$options['" + "#{site}'] " + "= #{value}"
eval(cmd)
end
end
#
# In : arg - argument list. i.e ARGV
# $options - loaded w/ default value
# Out : $options - those to execute are set to true
#
def checkParam(arg)
doAll = 1 #assume all need to do
index = 0
setAllOptions(false)
#process options
OptionParser.new do |opts|
opts.banner = "Usage: ruby auto.rb [-d LEVEL] [-j] [-f] [-e] [-u] [-s]
LEVEL defaults to 0, which means debug off
-j JK
-f Fisher
-e Eyny
-u UN BBS
-s 85 ST
when none is provided, all sites visited"
opts.on( '-d', '--debug level', 'debug level') { |level| $options['debug'] = level.to_i }
opts.on( '-j', '--jk', 'JK forum') {$options['doJK'] = true}
opts.on( '-f', '--fish', 'Fisher forum') {$options['doFish'] = true}
opts.on( '-e', '--eyny', 'EYNY forum') {$options['doEyny'] = true}
opts.on( '-u', '--un', 'UNBBS forum') {$options['doUn'] = true}
opts.on( '-s', '--st', '85ST forum') {$options['do85ST'] = true}
opts.on('-h', '--help', 'Display usage') {
puts "#{opts.banner}"
exit
}
end.parse arg
$allSites.keys.each do |site|
varName = "\$options['" +"#{site}']"
index += 1
dbg(" user #{varName}: #{eval(varName)}")
if eval(varName) then doAll = 0 end #once any is set, not all is requested
end
if doAll > 0 then
dbg("No flag found and to modify enable all options !", 2)
setAllOptions(true)
end
end
#
# Do jobs on $options that is true
#
def jobDispatcher()
$allSites.each do |site, link|
optName = "\$options['" + "#{site}']"
if eval(optName) then
dbg("#{site} w/ #{link}", 3)
cmd = "#{site}\(\"#{link}\")"
eval(cmd)
end
end
end
def doEyny(link)
bw = Watir::Browser.new :chrome
bw.goto link
div1 = bw.body.div(:id => 'wp').div(:class => 'y')
if div1 == NIL then
puts "No 'body.div.div.div' found"
return
end
span = div1.span(:class => 'pipe')
if span == NIL then
puts "No 'body.div.div.div.span' found"
return
end
span.hover
span1 = span.element(:xpath => './following-sibling::*[3]')
if span1 == NIL then
puts "No 'body.div.div.div.span1' found"
return
end
#puts "#{span1.inspect}, #{span1.text}"
span1.click
bw.div(:class => 'rfm').wait_until_present
#user name in a div
div = bw.div(:class => 'rfm')
tr = div.table.tbody.tr
span = tr.th.span
hashid = span.a.attribute_value("id")
hashid = hashid.sub("loginfield_","")
hashid = hashid.sub("_ctrl","")
nameid = "username_" << hashid
#(loginfield_)(.*)(_ctrl)
tr.text_field(:id => nameid).set $myID
#passwd is sibling of user name
div1 = div.element(:xpath => './following-sibling::*')
nameid = "password3_" << hashid
tr = div1.table.tbody.tr
tr.text_field(:id => nameid).set $myPW
bw.send_keys :enter
end
def doUn(link)
bw = Watir::Browser.new :chrome
bw.goto link
bw.div(:class => 'mb_1 mb_11').a.click
bw.div(:class => 'rfm').wait_until_present
#user name in a div
div = bw.div(:class => 'rfm')
tr = div.table.tbody.tr
label = tr.th.label
hashid = label.attribute_value("for")
#puts "#{label.inspect}, #{label.text} #{label.attribute_value("for")}"
tr.text_field(:id => hashid).set $myID
#passwd is sibling of user name
div1 = div.element(:xpath => './following-sibling::*')
tr = div1.table.tbody.tr
label = tr.th.label
hashid = label.attribute_value("for")
tr.text_field(:id => hashid).set $myPW
bw.send_keys :enter
end
def doCommon(link)
bw = Watir::Browser.new :chrome
bw.goto link
bw.text_field(:id => 'ls_username').set $myID
bw.text_field(:id => 'ls_password').set $myPW
bw.send_keys :enter
#bw.button(:class => 'pn vm').click
end
def doFish(link)
bw = Watir::Browser.new :chrome
bw.goto link
bw.div(:id => 'umenu').as[1].click
bw.text_field(:name => 'username').set $myID
bw.text_field(:name => 'password').set $myPW
bw.send_keys :enter
end
def doJK(link)
doCommon(link)
end
def do85ST(link)
bw = Watir::Browser.new :chrome
bw.goto link
bw.div(:class => 'logintx').as[0].click
bw.text_field(:name => 'username').set $myID
bw.text_field(:name => 'password').set $<PASSWORD>
bw.send_keys :enter
end
###
# main
checkParam(ARGV)
jobDispatcher
exit
doFish
doEyny
doUN
doJK($jkLink)
doJK($st85Link)
<file_sep>#!/bin/bash
#
# $1 : top directory to check CVS with
#
# change the [NEW_IP] in [FILE]
# $1 [FILE] file name, no check FILE here
# $2 [NEW_IP] to replace manuchen@xxx:/wincvs in [FILE], no check NEW_IP here
chgIPofFile() {
local FILE=$1
local NEW_IP=$2
# echo FILE=$FILE, NEW_IP=$NEW_IP
cat $FILE | sed -e "s/[0-9]\+\.[0-9]\+\.[0-9]\+\.[0-9]\+/$NEW_IP/" > $FILE.tmp
mv $FILE.tmp $FILE
}
############
### main ###
############
# 1. check top directory
test ! -d "$1" && echo Please input valid dir name && exit
TOP_DIR=$1
# 2. get cvs server IP
echo -n "Please input IP of CVS server: "
read NEW_IP
# 3. check cvs server IP
if [ ! -z "$NEW_IP" ]; then
TEST_IP=`echo $NEW_IP | grep -e "[0-9]\+\.[0-9]\+\.[0-9]\+\.[0-9]\+"`
if [ -z "$TEST_IP" ]; then
echo "Invalid IP ($NEW_IP)"
exit
fi
fi
echo Replacing new ip $NEW_IP
# 4. process replacement
find $TOP_DIR -path "*/CVS/Root" | while read f; do chgIPofFile $f $NEW_IP; done
<file_sep>#!/bin/bash
SPEED=100
test $# -ne 0 && SPEED=$1
echo Writing fan speed $SPEED
su -c "echo $SPEED >/sys/devices/pwm-fan/target_pwm"
<file_sep># need to copy ../qmake.conf to jetson-tx1-g++
#export ROOTFS=~/cross/nano_441
#export TARGET_ROOTFS=${ROOTFS}
#export CROSS_COMPILE=aarch64-linux-gnu-
#export PATH=~/bin/gcc-linaro-7.3.1-2018.05-x86_64_aarch64-linux-gnu/bin:${PATH}
#qmake gen makefile
# --sysroot="compile-time $ROOTFS"
# -rpath-link,
./configure -shared -c++std c++14 -opensource -release -recheck-all --confirm-license -device linux-jetson-tx1-g++ -device-option CROSS_COMPILE=${CROSS_COMPILE} -extprefix ${ROOTFS}/usr/local/qt5 -hostprefix /home/manu/cross/hostqt5 -skip webview -skip qtwebengine -opengl es2 -sysroot ${ROOTFS}
<file_sep>#!/bin/bash
echo "\$0=$0"
echo "\$ZSH_NAME=$ZSH_NAME"
echo "\$BASH_SOURCE=$BASH_SOURCE"
echo "\$PWD=$PWD"
echo ""
echo "Current directory is $PWD"
tmpDir=`readlink -f $PWD`
parentDir=`dirname $tmpDir`
pushd .. >/dev/null
echo "Parent directory is $PWD"
popd >/dev/null
tmpDir=`readlink -f $BASH_SOURCE`
parentDir=`dirname $tmpDir`
echo "Script directory is $parentDir"
<file_sep>#!/bin/bash
# put all files in a directory in host to a directory of adb device
#
# Usage:
# adb_mput.sh LOCAL_DIR REMOTE_DIR
# adb mput.sh FLIST REMOTE_DIR
# FLIST - file contains a list of files
#
usage() {
echo "Usage: adb_mput.sh LOCAL_DIR REMOTE_DIR"
echo " adb mput.sh FLIST REMOTE_DIR"
echo " FLIST - file contains a list of files"
}
############
### main ###
############
test $# -ne 2 && usage && exit
FLIST=
if [ -d "$1" ]; then
FLIST=/tmp/flist_mput.txt
ls $1 > $FLIST
else
test ! -f "$1" && usage && exit
FLIST=$1
fi
RDIR=$2
#awk '{system("adb push "$0 " /sdcard/Movies")}' $FLIST
for f in `cat $FLIST`; do
adb push $f $RDIR
done
<file_sep># Download base image jenkins:jenkins x64
# docker build -t manu-jenkins:0000 .
# RUN:
# docker run -d --name mj --user root -p 8080:8080 -p 50000:50000 -v /var/run/docker.sock:/var/run/docker.sock manu-jenkins:0000
# Check password: in /var/jenkins_home/secrets/initialAdminPassword
# docker start mj ... if ever powered off
# docker exec -it mj /bin/bash
# 00 is base
# must have USER root to avoid apt-key or something fail
# java -jar jenkins-cli.jar -auth manu:manu -s http://localhost:8080 get-job <myjob> > myjob.xml
# java -jar jenkins-cli.jar -auth manu:manu -s http://localhost:8080 create-job <myjob> < myjob.xml
FROM jenkins/jenkins:latest
LABEL maintainer="<EMAIL>"
LABEL version="00.00"
LABEL description="base of jenkins"
USER root
ENV TZ=Asia/Taipei
ENV APP="apt-transport-https ca-certificates curl gnupg2 software-properties-common"
RUN ln -snf /usr/share/zoneinfo/$TZ /etc/localtime && echo $TZ > /etc/timezone
RUN apt-get update && apt-get -y install $APP && curl -fsSL https://download.docker.com/linux/$(. /etc/os-release; echo "$ID")/gpg > /tmp/dkey; apt-key add /tmp/dkey && add-apt-repository "deb [arch=amd64] https://download.docker.com/linux/$(. /etc/os-release; echo "$ID") $(lsb_release -cs) stable" && apt-get update && apt-get -y install docker-ce
<file_sep>#!/bin/sh
#
# output error message and usage before end process
# In : $1- message
#
function usage()
{
test ! -z "$1" && echo "Error: $1"
echo "Usage: $0 SRC_KERNEL_TREE [TGT_KERNEL_TREE]"
echo " copy dvb-core, dvb-usb and frontends headers from SRC to TGT kernel tree"
echo " SRC_KERNEL_TREE: the source of root of kernel tree"
echo " TGT_KERNEL_TREE: the target of root of target tree,"
echo " if missed, /lib/modules/\`uname -r\`/source is used"
exit 1
}
COMMON_PATH=drivers/media/dvb
SUB_DIRS="$COMMON_PATH/dvb-core $COMMON_PATH/dvb-usb $COMMON_PATH/frontends"
TGT_KERNEL_ROOT=/lib/modules/`uname -r`/source
###################################
##### check source directory #####
test $# -lt 1 && usage "Incorrect arguments"
SRC_KERNEL_ROOT=$1
test ! -d "$SRC_KERNEL_ROOT" && usage "Missing source directory"
echo " SOURCE: $SRC_KERNEL_ROOT"
###################################
##### check target directory #####
shift
test ! -z "$1" && set TGT_KERNEL_ROOT=$1
test ! -d "$TGT_KERNEL_ROOT" && usage "Missing target directory"
echo " TARGET: $TGT_KERNEL_ROOT"
###########################################
##### processing each sub-directories #####
for dir in $SUB_DIRS; do
if [ ! -d $SRC_KERNEL_ROOT/$dir ]; then
echo "Missing source $SRC_KERNEL_ROOT/$dir ...skip"
continue
fi
if [ ! -d $TGT_KERNEL_ROOT/$dir ]; then
echo "Missing target $TGT_KERNEL_ROOT/$dir ...skip"
continue
fi
echo " copying $dir"
cp $SRC_KERNEL_ROOT/$dir/*.h $TGT_KERNEL_ROOT/$dir
done
<file_sep>#!/bin/bash
#for host
#
#sudo apt-get install virt-manager libvirt-bin qemu-system qemu-kvm linux-image-extra-virtual
#sudo echo 'auto eth0' >>/etc/network/interfaces
#sudo echo 'iface eth0 inet manual' >>/etc/network/interfaces
#sudo echo 'auto br0' >>/etc/network/interfaces
#sudo echo 'iface br0 inet dhcp' >>/etc/network/interfaces
#sudo echo '\tbridge_ports eth0' >>/etc/network/interfaces
#sudo echo '\tbridge_stp off' >>/etc/network/interfaces
#sudo echo '\tbridge_fd 0' >>/etc/network/interfaces
#sudo echo '\tbridge_maxwait 0' >>/etc/network/interfaces
sudo apt-get install -y xfce4 xfce4-terminal minicom nfs-common nfs-kernel-server
sudo apt-get install -y filezilla vsftpd tftpd-hpa g++ vim git gitk\
synaptic ssh meld dos2unix
sudo echo '/home/aten-3a00/ti *(rw,sync,no_subtree_check)' >>/etc/exports
echo 'export EDITOR=vim' >>~/.bashrc
<file_sep># almost follow chinese way (no KHR) but I also create symblink as western way
# sudo apt install symlinks nfs-kernel-server
# sudo vi /etc/exports:
# <add> / *(ro,fsid=root,no_root_squash,nohide,insecure,no_subtree_check)
# sudo exportfs -a
# sudo symlinks -c -r /
# delete config.cache
#test this
./configure -shared -c++std c++14 -opensource -release -recheck-all --confirm-license\
-device linux-jetson-tx1-g++ -device-option CROSS_COMPILE=aarch64-linux-gnu-\
-sysroot / -nomake tests -prefix /usr/local/qt5 -extprefix /usr/local/qt5 -hostprefix /usr/local/qt5 -skip webview -skip qtwebengine -opengl es2
#works
./configure -shared -c++std c++14 -opensource -release -recheck-all --confirm-license -device linux-jetson-tx1-g++ -device-option CROSS_COMPILE=/home/manu/bin/gcc-linaro-6.5.0-2018.12-x86_64_aarch64-linux-gnu/bin/aarch64-linux-gnu- -sysroot /home/manu/jetson -nomake examples -nomake tests -prefix /usr/local/qt5 -extprefix /home/manu/Downloads/qt-everywhere-src-5.14.1/NanoR32.3.1/qt5 -hostprefix /home/manu/Downloads/qt-everywhere-src-5.14.1/NanoR32.3.1/qt5-host -skip webview -skip qtwebengine -opengl es2
#not this
./configure -shared -c++std c++14 \
-opensource -release --confirm-license -no-pkg-config \
-device linux-jetson-tx1-g++ \
-device-option CROSS_COMPILE=/home/manu/bin/gcc-linaro-6.5.0-2018.12-x86_64_aarch64-linux-gnu/bin/aarch64-linux-gnu- \
-sysroot /home/manu/jetson \
-nomake examples -nomake tests \
-prefix /usr/local/qt5 \
-extprefix /home/manu/Downloads/qt-everywhere-src-5.14.1/NanoR32.3.1/qt5 \
-hostprefix /home/manu/Downloads/qt-everywhere-src-5.14.1/NanoR32.3.1/qt5-host \
-skip webview -skip qtwebengine -opengl es2
./configure -shared -c++std c++14 \
-opensource -release --confirm-license -no-pkg-config \
-device linux-jetson-tx1-g++ \
-device-option CROSS_COMPILE=/home/manu/bin/gcc-linaro-6.5.0-2018.12-x86_64_aarch64-linux-gnu/bin/aarch64-linux-gnu- \
-sysroot /home/manu/Downloads/nano-rootfs \
-nomake examples -nomake tests \
-prefix /usr/local/qt5 \
-extprefix /home/manu/Downloads/qt-everywhere-src-5.14.1/JetsonNano/qt5 \
-hostprefix /home/manu/Downloads/qt-everywhere-src-5.14.1/JetsonNano/qt5-host \
-skip webview -skip qtwebengine -opengl es2
./configure -shared -c++std c++14 \
-opensource -release --confirm-license -no-pkg-config \
-device linux-jetson-tx1-g++ \
-device-option CROSS_COMPILE=/home/manu/bin/gcc-linaro-5.5.0-2017.10-x86_64_aarch64-linux-gnu/bin/aarch64-linux-gnu- \
-sysroot /home/manu/Downloads/nano-rootfs \
-nomake examples -nomake tests \
-prefix /usr/local/qt5 \
-extprefix /home/manu/Downloads/qt-everywhere-src-5.14.1/JetsonNano/qt5 \
-hostprefix /home/manu/Downloads/qt-everywhere-src-5.14.1/JetsonNano/qt5-host \
-skip webview -skip qtwebengine -opengl es2
<file_sep>#!/bin/bash
# Usage: new_install manuchen
#ubuntu install mfx
#sudo apt install -y libmfx1 intel-media-va-driver-non-free libigfxcmrt7 libva-drm2 libva-x11-2 libmfx-dev libmfx-tools
#sudo apt install -y gstreamer1.0-plugins-base gstreamer1.0-plugins-good gstreamer1.0-plugins-bad gstreamer1.0-plugins-ugly gstreamer1.0-libav gstreamer1.0-tools
#git clone https://github.com/intel/gstreamer-media-SDK.git
#sudo apt install cmake autoconf libtool libglib2.0-dev libudev-dev libgstreamermm-1.0-dev libva-dev libdrm-dev libgst-dev libgstreamer-plugins-*-dev
#mkdir build && cd build && cmake ..
#make; make install
#
# in : S1 - user name to set permision with
#
get_param()
{
if [ -z "$1" ]; then
G_USER=`whoami`
else
G_USER=$1
fi
X64_CPU=`uname -m`
MY_UID=`id $G_USER | cut -d"=" -f2 | cut -d"(" -f1`
test -z "$MY_UID" && exit
echo G_USER = $G_USER, X64_CPU = $X64_CPU, MY_UID = $MY_UID
}
#
# get answer among (y/n/s)
# in : $1 - string to highligh for user
# out : g_ANS = 'n' or 's' or default = 'y'
#
get_answer()
{
MESSAGE=$1
echo -en "\\033[0;33m"
echo -en "$MESSAGE (Yes/No/Skip): "
echo -en "\\033[0;39m"
read ans
if [ $ans == 'N' -o $ans == 'n' ]; then
g_ANS='n'
elif [ $ans == 'S' -o $ans == 's' ]; then
g_ANS='s'
else
g_ANS='y'
fi
}
#
# setting .bashrc
#
do_bashrc()
{
if `grep EDITOR ~/.bashrc`; then
get_answer "The bashrc might be updated, continue to process ?"
if [ $g_ANS == 'n' ]; then
exit 0
elif [ $g_ANS == 's' ]; then
return
fi
fi
##### CVS setting #####
#echo "export CVSROOT=:pserver:[email protected]:/home/cvsroot" >> ~/.bashrc
#echo "export EDITOR=vim" >> ~/.bashrc
##### CIFS setting #####
#echo -n "alias lnaver='sudo mount.cifs //avermedia.com/NETLOGON /mnt/aver" >> ~/.bashrc
#echo -n " -o uid=$MY_UID,username=avermedia\\" >> ~/.bashrc
#echo "\\\\a000989'" >> ~/.bashrc
#echo -n "alias lndriver='sudo mount.cifs //10.1.9.247/Driver /mnt/driver" >> ~/.bashrc
#echo -n " -o uid=$MY_UID,username=avermedia\\" >> ~/.bashrc
#echo "\\\\a000989'" >> ~/.bashrc
#echo -n "alias ln618='sudo mount.cifs //10.1.9.247/b618 /mnt/618" >> ~/.bashrc
#echo -n " -o uid=$MY_UID,username=avermedia\\" >> ~/.bashrc
#echo "\\\\a000989'" >> ~/.bashrc
#echo -n "alias lngit='sudo mount.cifs //10.1.9.247/vl/GIT /mnt/git" >> ~/.bashrc
#echo -n " -o uid=$MY_UID,username=avermedia\\" >> ~/.bashrc
#echo "\\\\a000989'" >> ~/.bashrc
#echo -n "alias ln200='sudo mount.cifs //10.1.9.200/manuchen /mnt/200" >> ~/.bashrc
#echo " -o username=manuchen'" >> ~/.bashrc
#echo -n "alias lnsrc='sudo mount.cifs //10.1.9.200/Resources /mnt/src" >> ~/.bashrc
#echo " -o username=manuchen'" >> ~/.bashrc
#ubuntu 21.10 needs forceuid,forcegid
echo "alias smbnas='sudo mount.cifs //192.168.3.11/share/git ~/nas -o forceuid,forcegid,uid=\$(id -u),gid=\$(id -g),username=manu,vers=1.0'" >> ~/.bashrc
echo "export EDITOR=vim" >> ~/.bashrc
#echo "alias goshare='cd /media/sf_share'" >> ~/.bashrc
}
#
# check if application installed well
#
check_app()
{
CHECKLIST="gitk cifs-utils vim ssh minicom g++ build-essential terminator gparted chromium-browser fcitx-libs-dev fcitx-tools fcitx-table cmake"
PURGE_LIST="libreoffice-* firefox"
#CHECKLIST="cvs git tftp mount.cifs vim ssh kaffeine vlc mplayer minicom v4l2ucp g++ codeblocks"
for app in $CHECKLIST; do
test ! `which $app` && echo "$app is not installed !!!"
done
}
#
# install applications
#
do_app_install()
{
#front type freemono 14
BASE_APP="gitk vim terminator build-essential curl wget cifs-utils"
# dayi for ubuntu
# 1. setup language: install chinese language and logout and login
# 2. follow
# https://github.com/Alger23/ubuntu_dayi_for_ibus
# 3. setup keyboard: add chinese/dayi input source
# use ssh-keygen and register id_rsa.pub to github
# git clone <EMAIL>:twManu/shell.git
#APPLIST="cvs git-core nfs-kernel-server tftpd-hpa tftp-hpa smbfs vim ssh samba \
# gitk kaffeine xine-ui vlc mplayer minicom v4l2ucp g++ filezilla codeblocks \
# wireshark gnupg flex bison gperf build-essential zip curl zlib1g-dev libc6-dev \
# x11proto-core-dev libx11-dev libgl1-mesa-dev g++-multilib mingw32 tofrodos \
# python-markdown libxml2-utils xsltproc"
APPLIST=${BASEAPP}
test $X64_CPU = "x86_64" && APPLIST="$APPLIST ia32-libs lib32ncurses5-dev lib32z1-dev lib32readline-gplv2-dev"
if [ ! -z `which cvs` ] ; then
get_answer "The CVS is installed, continue to process ?"
if [ $g_ANS == 'n' ]; then
exit 0
elif [ $g_ANS == 's' ]; then
return
fi
fi
#wget https://dl.google.com/linux/direct/google-chrome-stable_current_amd64.deb
sudo apt-get -y install $APPLIST
check_app
cat >~.vimrc <<EOF
syntax enable
set smartindent
set tabstop=4
set shiftwidth=4
set noexpandtab
EOF
}
do_mnt()
{
DIRLIST="/mnt/aver /mnt/driver /mnt/git"
for dir in $DIRLIST; do
if [ ! -e $dir ]; then
sudo mkdir $dir
else
echo "$dir exists...skip creating directory" > /dev/null
fi
done
}
#
# configure samba
# in : $1 - username
#
do_smb()
{
SYSSMBCFG=/etc/samba/smb.conf
SMBCFG=./tmp.conf
if grep $G_USER $SYSSMBCFG; then
get_answer "The $G_USER is present in smb.conf, continue to process ?"
if [ $g_ANS == 'n' ]; then
exit 0
elif [ $g_ANS == 's' ]; then
return
fi
fi
if [ -f $SYSSMBCFG ]; then
cp $SYSSMBCFG $SMBCFG
echo "" >> $SMBCFG
echo "[$G_USER]" >> $SMBCFG
echo " path = /home/$G_USER/" >> $SMBCFG
echo " browseable = yes" >> $SMBCFG
echo " read only = no" >> $SMBCFG
echo " guest ok = no" >> $SMBCFG
echo " create mask = 0664" >> $SMBCFG
echo " directory mask = 0755" >> $SMBCFG
echo " force user = $G_USER" >> $SMBCFG
echo " force group = $G_USER" >> $SMBCFG
sudo mv $SMBCFG $SYSSMBCFG
fi
if [ ! -z `which smbpasswd` ]; then
sudo smbpasswd -L -a $G_USER
sudo smbpasswd -L -e $G_USER
fi
}
echoCmd() {
[ -n "$1" ] && {
echo "$1"
eval "$1"
}
}
#since alias takes no parameter, use function instead
ssh20() {
doDel=
NET=192.168.20
if [ $# -lt 1 ]; then
echo Usage: ssh20 LAST_IP [USER]
echo " ssh root@${NET}.LAST_IP by default"
else
USER=root
[ -n "$2" -a "$2" == "-d" ] && doDel=yes
if [ -n "${doDel}" ]; then
# try to kill existing ssh end with $1
curSSH=`ps aux | grep ssh | egrep "$1\$" | awk '{ print $2 }'`
curSSHcount=`echo ${curSSH} | wc -l`
echo "found ${curSSHcount} ssh process:"
for line in ${curSSH}; do
id=`echo ${line} | sed -e s/[^0-9]//g`
echoCmd "kill -9 ${id}"
done
fi
ssh-keygen -f "/home/oem/.ssh/known_hosts" -R "${NET}.$1"
echoCmd "ssh ${USER}@${NET}.$1"
fi
}
#NOTE:
#1.the mount.cifs uid=<uid#>, the user name becames unavailable after 11.10
#2.the ia32-libs fails x86 installation
get_param $1
do_app_install
#do_mnt
do_bashrc
#do_smb
<file_sep>#!/bin/sh
# there are 37 channels in area of post code 95035
TMP_FILE=tmp.log
grep "SignalLocked =" scan.LOG >$TMP_FILE
cat $TMP_FILE | awk 'BEGIN { x=0 } {print "channel"x+1 $4,$5; ++x; x%=37 }'
<file_sep>#!/bin/bash
# demonstrate usage of named pipe
#
# Usage:
# named_pipe [-s|-c] [-m MSG]
#
usage() {
echo "USage: named_pipe [-s|-c] [-m MSG]"
echo " -s: run as a server"
echo " -c: run as a client"
echo " MSG: client message to display in server side"
echo " quit mean to stop server"
}
pipe=/tmp/testpipe
g_server=0
g_msg=
#
# Update g_xxx variable
#
parse_param() {
while getopts ":scm:" opt; do
case $opt in
s) g_server=1;;
c) g_server=0;;
m) g_msg=$OPTARG;;
\?) usage
exit;;
esac
done
OPTIND=1
echo "g_server = $g_server, g_msg = $g_msg"
}
#
# server code: read from pipe
#
do_server() {
test ! -p $pipe && mkfifo $pipe
trap "rm -f $pipe" EXIT
while true; do
if read line <$pipe; then
if [[ "$line" == 'quit' ]]; then
break
fi
echo $line
fi
done
echo "Reader exiting"
}
#
# client code: write to pipe
#
do_client() {
if [[ ! -p $pipe ]]; then
echo "Reader not running"
exit 1
fi
if [[ ! -z "$g_msg" ]]; then
echo $g_msg >$pipe
else
echo "Hello from $$" >$pipe
fi
}
############
### main ###
############
parse_param $@
if [[ $g_server -eq 1 ]]; then
do_server
else
do_client
fi
<file_sep>ls -l | grep -e '^d' | awk '{print $9}'
<file_sep>#!/usr/bin/ruby
# encoding: UTF-8
taiwan = [
'http://stockq.org/index/TWSE.php'\
, '$TWII - SharpCharts Workbench - StockCharts.com'
]
shanghai = [
'http://stockq.org/index/SHCOMP.php'\
, '$SSEC - SharpCharts Workbench - StockCharts.com'
]
japan = [
'http://stockq.org/index/NKY.php'\
, '$NIKK - SharpCharts Workbench - StockCharts.com'
]
dutch = [
'http://stockq.org/index/DAX.php'\
, '$DAX - SharpCharts Workbench - StockCharts.com'
]
dow = [
'http://stockq.org/index/INDU.php'\
, '$INDU - SharpCharts Workbench - StockCharts.com'
]
nasdaq = [
'http://stockq.org/index/CCMP.php'\
, '$COMPQ - SharpCharts Workbench - StockCharts.com'
]
markets = [ taiwan, shanghai, japan, dutch, dow, nasdaq ]
require 'watir-webdriver'
#give options default value
$options = {'debug' => 0}
#
# output message if message level is no less than current setting
#
def dbg(msg, msgLevel = 1)
if $options['debug'] >= msgLevel then
puts "#{msg}"
end
end
def fire(location)
bw = Watir::Browser.new :chrome
bw.goto location[0]
b = bw.b(:text => '技術線圖')
button = b.parent.elements[1]
button.click
bw.window(:title => location[1]).wait_until_present
bw.window(:title => location[1]).use do
bw.text_field(:id => 'overArgs_0').wait_until_present
bw.text_field(:id => 'overArgs_0').set 5
bw.text_field(:id => 'overArgs_1').set 22
bw.text_field(:id => 'overArgs_2').set 66
bw.input(:value => 'Update').click
end
end
###
# main
markets.each { |place| fire place }
<file_sep>#!/bin/sh
#
# In : $1- message
#
function usage()
{
test ! -z "$1" && echo "Error: $1"
echo " Usage: $0 FILE"
echo " To remove comments in FILE"
exit 0
}
#
# main
#
# check parameter count & file existence
#
test $# != 1 && usage "Incorrect number of parameter !!!"
test ! -f $1 && usage "$1 doesn't exist !!!"
cpp -fpreprocessed $1 >$1.pre
diff $1 $1.pre >$1.diff
cat $1.diff | grep -e '<[ tab]*#[ tab]*define' | grep -v -e '//' |\
sed 's/^<[ tab]*#[ tab]*define/#define/g' >$1.define
<file_sep>#!/bin/bash
# remove ^M in given file
#
# Usage:
# removeUP_M.sh [-h] [-f FILE] -n
#
usage() {
echo "Usage: removeUP_M.sh [-h] [-n] -f FILE"
echo " -n: replace ^M with '\n'"
echo " FILE: input file to work with"
}
g_newline=0
g_file=
#
# Update g_xxx variable
#
parse_param() {
while getopts ":nf:" opt; do
case $opt in
n) g_newline=1;;
f) g_file=$OPTARG;;
\?) usage
exit;;
esac
done
OPTIND=1
#echo "g_newline = $g_newline"
}
#
# validate parameter
#
check_param() {
test -z "$g_file" && usage && exit 1
if [[ ! -f $g_file ]]; then
echo "Not being able to process $g_file"
exit 1
fi
}
#
# process file replacement
#
do_action() {
#cat cx231xx-audio.c | sed "s/\x0d/\\n/g" >cx231xx-audio1.c
local tmp_file=/tmp/tmpfile_removeUP_M
local process=0
echo " ### first few lines of $g_file follows ###"
cat $g_file > $tmp_file
head -n 7 $tmp_file
echo
echo -n "To process $g_file "
test $g_newline -eq 1 && echo -n "w/ new-line replacement "
echo -n "(y/n) ? "
read ans
case $ans in
y*|Y*) process=1;;
*) echo "...stop processing";;
esac
test $process -eq 0 && exit 1
mv $g_file $g_file.bak
if [[ $g_newline -eq 1 ]]; then
cat $g_file.bak | sed "s/\x0d/\\n/g" > $g_file
else
cat $g_file.bak | sed "s/\x0d//g" > $g_file
fi
}
############
### main ###
############
parse_param $@
check_param
do_action
<file_sep>#!/bin/bash
OK=0
CANCEL=1
ESC=255
BACKTITLE="Cody Blog - Example"
Menu(){
while :
do {
Selection=$(dialog --title "CodyBlog - Menu" --clear \
--backtitle "$BACKTITLE" \
--cancel-label "Exit" \
--menu "Choose one" 12 45 5 \
one "Test 01" \
two "Test 02" \
2>&1 > /dev/tty)
result=$?
if [ $result -eq $OK ]; then
Exit "$Selection selected !"
elif [ $result -eq $CANCEL ] || [ $result -eq $ESC ]; then
Exit
fi
} done
}
Exit(){
clear
if [ -z "$1" ]; then
echo "Program Terminated."
else
echo $1
fi
exit
}
Menu
| e4d02e117c5021a4848e6fe2f89a7caf3cea80a4 | [
"Python",
"Ruby",
"Dockerfile",
"Shell"
] | 51 | Python | twManu/shell | 1e591a178f1f5cb54c23e9b25de45af68ba2cf56 | 6d8019f5ca0432aec3c116f177434a4edbe69587 | |
refs/heads/main | <repo_name>EbarriosCode/BackendDotnet_CleanArchitecture<file_sep>/Countries.Infra.Data/Data/DbInitializer.cs
using Countries.Infra.Data.DataContext;
using Countries.Models.Models;
using Microsoft.EntityFrameworkCore;
using Microsoft.Extensions.DependencyInjection;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Security.Cryptography.X509Certificates;
using System.Text;
using System.Threading.Tasks;
namespace Countries.Infra.Data.Data
{
public static class DbInitializer
{
public static void Initialize(IServiceProvider serviceProvider)
{
using (var _context = new CountriesDbContext(serviceProvider.GetRequiredService<DbContextOptions<CountriesDbContext>>()))
{
// Add Countries in DB
if (_context.Countries.Any())
return;
_context.Countries.AddRange(
new Country { Name = "Francia", Alpha_2 = "Fr", Alpha_3 = "Fra", NumericCode = "250" },
new Country { Name = "Alemania", Alpha_2 = "De", Alpha_3 = "Deu", NumericCode = "276" },
new Country { Name = "Guatemala", Alpha_2 = "Gt", Alpha_3 = "Gtm", NumericCode = "320" }
);
_context.SaveChanges();
// Add Subdivision in Db
if (_context.Subdivisions.Any())
return;
_context.Subdivisions.AddRange(
// Francia
new Subdivision { Name = "French Polynesia", CountryID = _context.Countries.FirstOrDefault(c => c.Alpha_2.Contains("Fr")).CountryID },
new Subdivision { Name = "Saint Barthélemy", CountryID = _context.Countries.FirstOrDefault(c => c.Alpha_2.Contains("Fr")).CountryID },
new Subdivision { Name = "Saint Martin", CountryID = _context.Countries.FirstOrDefault(c => c.Alpha_2.Contains("Fr")).CountryID },
new Subdivision { Name = "Saint Pierre and Miquelon", CountryID = _context.Countries.FirstOrDefault(c => c.Alpha_2.Contains("Fr")).CountryID },
new Subdivision { Name = "Wallis and Futuna", CountryID = _context.Countries.FirstOrDefault(c => c.Alpha_2.Contains("Fr")).CountryID },
// Alemania
new Subdivision { Name = "Baden-Württemberg", CountryID = _context.Countries.FirstOrDefault(c => c.Alpha_2.Contains("De")).CountryID },
new Subdivision { Name = "Bavaria (Bayern)", CountryID = _context.Countries.FirstOrDefault(c => c.Alpha_2.Contains("De")).CountryID },
new Subdivision { Name = "Berlin", CountryID = _context.Countries.FirstOrDefault(c => c.Alpha_2.Contains("De")).CountryID },
new Subdivision { Name = "Brandenburg", CountryID = _context.Countries.FirstOrDefault(c => c.Alpha_2.Contains("De")).CountryID },
new Subdivision { Name = "Bremen", CountryID = _context.Countries.FirstOrDefault(c => c.Alpha_2.Contains("De")).CountryID },
// Guatemala
new Subdivision { Name = "Suchitepequez", CountryID = _context.Countries.FirstOrDefault(c => c.Alpha_2.Contains("Gt")).CountryID },
new Subdivision { Name = "<NAME>", CountryID = _context.Countries.FirstOrDefault(c => c.Alpha_2.Contains("Gt")).CountryID },
new Subdivision { Name = "Quetzaltenango", CountryID = _context.Countries.FirstOrDefault(c => c.Alpha_2.Contains("Gt")).CountryID },
new Subdivision { Name = "Huehuetenango", CountryID = _context.Countries.FirstOrDefault(c => c.Alpha_2.Contains("Gt")).CountryID },
new Subdivision { Name = "Izabal", CountryID = _context.Countries.FirstOrDefault(c => c.Alpha_2.Contains("Gt")).CountryID }
);
_context.SaveChanges();
}
}
}
}
<file_sep>/Application/Services/CountriesService.cs
using Application.Helpers;
using Application.Interfaces;
using Countries.Common.Classes;
using Countries.Infra.Data.Repositories.Custom;
using Countries.Models.Models;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace Application.Services
{
public class CountriesService : ICountriesService
{
private readonly ICountriesRepository _repository;
private string includeProperties = string.Empty;
public CountriesService(ICountriesRepository repository) => this._repository = repository;
public async Task<PagedList<Country>> GetCountries(CountryParameters parameters)
{
try
{
var result = new List<Country>();
if (parameters != null)
{
#region Filter by Name or Alpha2 Code and Orderby Numeric Code
if (!string.IsNullOrEmpty(parameters.Name) && parameters.OrderByNumericCode)
result = this._repository.Get(x => x.Name.Contains(parameters.Name), x => x.OrderBy(y => y.NumericCode), includeProperties) as List<Country>;
else if (!string.IsNullOrEmpty(parameters.Name) && !parameters.OrderByNumericCode)
result = this._repository.Get(x => x.Name.Contains(parameters.Name), null, includeProperties) as List<Country>;
else if (!string.IsNullOrEmpty(parameters.Alpha2) && parameters.OrderByNumericCode)
result = this._repository.Get(x => x.Alpha_2.Contains(parameters.Alpha2), x => x.OrderBy(y => y.NumericCode), includeProperties) as List<Country>;
else if (!string.IsNullOrEmpty(parameters.Alpha2) && !parameters.OrderByNumericCode)
result = this._repository.Get(x => x.Alpha_2.Contains(parameters.Alpha2), null, includeProperties) as List<Country>;
else if (!string.IsNullOrEmpty(parameters.Name) && !string.IsNullOrEmpty(parameters.Alpha2) && parameters.OrderByNumericCode)
result = this._repository.Get(x => x.Name.Contains(parameters.Name) && x.Alpha_2.Contains(parameters.Alpha2), x => x.OrderBy(y => y.NumericCode), includeProperties) as List<Country>;
else if (!string.IsNullOrEmpty(parameters.Name) && !string.IsNullOrEmpty(parameters.Alpha2) && !parameters.OrderByNumericCode)
result = this._repository.Get(x => x.Name.Contains(parameters.Name) && x.Alpha_2.Contains(parameters.Alpha2), null, includeProperties) as List<Country>;
else
result = this._repository.Get(null, null, includeProperties) as List<Country>;
#endregion
}
return await Task.Run(() => PagedList<Country>.ToPagedList(result, parameters.PageNumber, parameters.PageSize));
}
catch (Exception)
{
throw;
}
}
public async Task<Country> CreateCountryAsync(Country country)
{
Country created = new();
try
{
if (country != null)
{
created = await this._repository.CreateAsync(country);
}
}
catch (Exception)
{
throw;
}
return created;
}
public Country GetCountry(int id)
{
Country result = null;
includeProperties = "Subdivisions";
try
{
List<Country> country = this._repository.Get(x => x.CountryID == id, null, includeProperties) as List<Country>;
if (country.Count > 0)
result = country[0];
}
catch (Exception)
{
throw;
}
return result;
}
public async Task<Country> UpdateCountryAsync(Country country)
{
Country updated = new();
try
{
if(country != null)
{
updated = await this._repository.UpdateAsync(country, country.CountryID);
}
}
catch (Exception)
{
throw;
}
return updated;
}
public async Task<int> DeleteCountryAsync(Country country)
{
int deleted = 0;
try
{
if (country != null)
deleted = await this._repository.DeleteAsync(country);
}
catch (Exception)
{
throw;
}
return deleted;
}
}
}
<file_sep>/Application/Interfaces/ISubdivisionsService.cs
using Application.Helpers;
using Countries.Common.Classes;
using Countries.Models.Models;
using System.Threading.Tasks;
namespace Application.Interfaces
{
public interface ISubdivisionsService
{
Task<PagedList<Subdivision>> GetSubdivisions(SubdivisionParameter parameters);
Subdivision GetSubdivision(int id);
Task<Subdivision> CreateSubdivisionAsync(Subdivision subdivision);
Task<Subdivision> UpdateSubdivisionAsync(Subdivision subdivision);
Task<int> DeleteSubdivisionAsync(Subdivision subdivision);
}
}
<file_sep>/Application/DTO/CountryDTO.cs
using Countries.Models.Models;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Application.DTO
{
public class CountryDTO : Country
{
}
}
<file_sep>/Countries.Infra.Data/Repositories/Custom/CountriesRepository.cs
using Countries.Infra.Data.Repositories.Generic;
using Countries.Models.Models;
namespace Countries.Infra.Data.Repositories.Custom
{
public interface ICountriesRepository : IBaseRepository<Country>
{
}
public class CountriesRepository : BaseRepository<Country>, ICountriesRepository
{
public CountriesRepository(IUnitOfWork _unitOfWork)
: base(_unitOfWork)
{ }
}
}
<file_sep>/Countries.Common/Classes/SubdivisionParameter.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Countries.Common.Classes
{
public class SubdivisionParameter : PagingParameters
{
}
}
<file_sep>/IoC/DependencyContainer.cs
using Application.Interfaces;
using Application.Services;
using Countries.Infra.Data.Repositories.Custom;
using Countries.Infra.Data.Repositories.Generic;
using Microsoft.Extensions.DependencyInjection;
namespace IoC
{
public static class DependencyContainer
{
public static IServiceCollection AddDependency(this IServiceCollection services)
{
// Inject the service generic repository
services.AddScoped<IUnitOfWork, UnitOfWork>();
// Inject the service JWT
services.AddTransient<IJWTService, JWTService>();
// Inject the service Countries
services.AddScoped<ICountriesService, CountriesService>();
services.AddScoped<ICountriesRepository, CountriesRepository>();
// Inject the service Subdivisions
services.AddScoped<ISubdivisionsService, SubdivisionsService>();
services.AddScoped<ISubdivisionsRepository, SubdivisionsRepository>();
return services;
}
}
}
<file_sep>/Countries.Models/Models/Subdivision.cs
using System.ComponentModel.DataAnnotations;
using System.ComponentModel.DataAnnotations.Schema;
namespace Countries.Models.Models
{
[Table("Subdivison")]
public class Subdivision
{
[Key]
public int SubdivisonID { get; set; }
[Required(ErrorMessage = "The field {0} is required")]
[StringLength(30)]
public string Name { get; set; }
public int CountryID { get; set; }
}
}
<file_sep>/Countries.Common/Resources/Literals.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Countries.Common.Resources
{
public class Literals
{
public static string URI_API_COUNTRIES = "api/Countries";
public static string URI_API_SUBDIVISIONS = "api/Subdivisions";
}
}
<file_sep>/Application/Interfaces/IJWTService.cs
using Countries.Common.Classes;
using System;
using System.Collections.Generic;
using System.IdentityModel.Tokens.Jwt;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Application.Interfaces
{
public interface IJWTService
{
Task<UserToken> BuildToken(UserInfo userInfo);
JwtSecurityToken DecodeJwt(string jwt);
}
}
<file_sep>/Countries.API/Startup.cs
using Countries.Infra.Data.DataContext;
using IoC;
using Microsoft.AspNetCore.Authentication.JwtBearer;
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.AspNetCore.Identity;
using Microsoft.EntityFrameworkCore;
using Microsoft.Extensions.Configuration;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Hosting;
using Microsoft.IdentityModel.Tokens;
using Microsoft.OpenApi.Models;
using Newtonsoft.Json;
using System;
using System.Text;
using System.Text.Json.Serialization;
using System.Threading.Tasks;
namespace Countries.API
{
public class Startup
{
public Startup(IConfiguration configuration)
{
Configuration = configuration;
}
public IConfiguration Configuration { get; }
// This method gets called by the runtime. Use this method to add services to the container.
public void ConfigureServices(IServiceCollection services)
{
// Add Entity Framework DbContext
services.AddDbContext<CountriesDbContext>(options => options.UseSqlServer(this.Configuration.GetConnectionString("DefaultConnection")));
services.AddControllersWithViews();
services.AddRazorPages();
// Indentity Server Configuration
services.AddIdentity<IdentityUser, IdentityRole>()
.AddRoles<IdentityRole>()
.AddEntityFrameworkStores<CountriesDbContext>()
.AddDefaultTokenProviders();
// Configuración JWT
services.AddAuthentication(x =>
{
x.DefaultAuthenticateScheme = JwtBearerDefaults.AuthenticationScheme;
x.DefaultChallengeScheme = JwtBearerDefaults.AuthenticationScheme;
}).AddJwtBearer(options =>
{
options.TokenValidationParameters = new TokenValidationParameters
{
ValidateIssuer = true,
ValidateAudience = true,
ValidateLifetime = true,
ValidateIssuerSigningKey = true,
ValidIssuer = Configuration["JwtIssuer"],
ValidAudience = Configuration["JwtAudience"],
IssuerSigningKey = new SymmetricSecurityKey(Encoding.UTF8.GetBytes(Configuration["JwtSecurityKey"])),
ClockSkew = TimeSpan.Zero
};
});
services.AddSwaggerGen(c =>
{
c.SwaggerDoc("v1", new OpenApiInfo
{
Title = "Countries.API",
Version = "v1" ,
Contact = new OpenApiContact
{
Name = "<NAME>",
Email = "dev_<EMAIL>",
Url = new Uri("https://twitter.com/ebarriosdev"),
}
});
});
// Init IoC Services
DependencyContainer.AddDependency(services);
// EnableCors
services.AddCors(policy =>
{
policy.AddPolicy("CorsPolicy", opt => opt
.AllowAnyOrigin()
.AllowAnyHeader()
.AllowAnyMethod()
.WithExposedHeaders("X-Pagination"));
});
}
private async Task CreateRoles(IServiceProvider serviceProvider)
{
var RoleManager = serviceProvider.GetRequiredService<RoleManager<IdentityRole>>();
bool adminRoleExists = await RoleManager.RoleExistsAsync("Admin");
if (!adminRoleExists)
await RoleManager.CreateAsync(new IdentityRole("Admin"));
}
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
public void Configure(IApplicationBuilder app, IWebHostEnvironment env, IServiceProvider services)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
app.UseSwagger();
app.UseSwaggerUI(c => c.SwaggerEndpoint("/swagger/v1/swagger.json", "Countries.API v1"));
}
JsonConvert.DefaultSettings = () => new JsonSerializerSettings
{
ReferenceLoopHandling = ReferenceLoopHandling.Ignore,
};
app.UseHttpsRedirection();
app.UseBlazorFrameworkFiles();
app.UseStaticFiles();
app.UseRouting();
app.UseCors(options => options.AllowAnyOrigin());
app.UseAuthentication();
app.UseAuthorization();
// Create Roles in Database
CreateRoles(services).Wait();
app.UseEndpoints(endpoints =>
{
endpoints.MapRazorPages();
endpoints.MapControllers();
endpoints.MapFallbackToFile("index.html");
});
}
}
}
<file_sep>/Countries.SPA/Services/CountriesHttpService.cs
using Application.DTO;
using Application.Helpers;
using Countries.Common.Classes;
using Countries.Common.Resources;
using Countries.SPA.Helpers.Paging;
using Microsoft.AspNetCore.WebUtilities;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Net.Http;
using System.Net.Http.Json;
using System.Text.Json;
using System.Threading.Tasks;
namespace Countries.SPA.Services
{
public interface ICountriesHttpService
{
Task<PagingResponse<CountryDTO>> GetCountriesDTOAsync(CountryParameters parameters);
Task<List<CountryDTO>> GetCountriesDTOAsync();
Task<CountryDTO> GetByID(int id);
Task<CountryDTO> Create(CountryDTO model);
Task<CountryDTO> Update(CountryDTO model);
Task<int> Delete(int id);
}
public class CountriesHttpService : ICountriesHttpService
{
private readonly HttpClient _client;
public CountriesHttpService(HttpClient client) => this._client = client;
public async Task<PagingResponse<CountryDTO>> GetCountriesDTOAsync(CountryParameters parameters)
{
var queryStringParam = new Dictionary<string, string>
{
["pageNumber"] = parameters.PageNumber.ToString()
};
var response = await _client.GetAsync(QueryHelpers.AddQueryString(Literals.URI_API_COUNTRIES, queryStringParam));
var content = await response.Content.ReadAsStringAsync();
if (!response.IsSuccessStatusCode)
{
throw new ApplicationException(content);
}
var pagingResponse = new PagingResponse<CountryDTO>
{
Items = JsonSerializer.Deserialize<List<CountryDTO>>(content, new JsonSerializerOptions { PropertyNameCaseInsensitive = true }),
MetaData = JsonSerializer.Deserialize<MetaData>(response.Headers.GetValues("X-Pagination").First(), new JsonSerializerOptions { PropertyNameCaseInsensitive = true })
};
return pagingResponse;
}
public async Task<List<CountryDTO>> GetCountriesDTOAsync()
{
var result = await this._client.GetFromJsonAsync<List<CountryDTO>>(Literals.URI_API_COUNTRIES);
return await Task.FromResult(result);
}
public async Task<CountryDTO> GetByID(int id)
{
var response = await this._client.GetFromJsonAsync<CountryDTO>($"{Literals.URI_API_COUNTRIES}/{id}");
return response;
}
public async Task<CountryDTO> Create(CountryDTO model)
{
// Insertar en el api
var response = await this._client.PostAsJsonAsync(Literals.URI_API_COUNTRIES, model);
if (response.StatusCode == HttpStatusCode.Created)
{
model = await response.Content.ReadFromJsonAsync<CountryDTO>();
return model;
}
else
{
string str = await response.Content.ReadAsStringAsync();
throw new Exception(str);
}
}
public async Task<CountryDTO> Update(CountryDTO model)
{
var response = await _client.PutAsJsonAsync(Literals.URI_API_COUNTRIES, model);
if (response.StatusCode == HttpStatusCode.OK)
{
model = await response.Content.ReadFromJsonAsync<CountryDTO>();
return model;
}
else
{
string str = await response.Content.ReadAsStringAsync();
throw new Exception(str);
}
}
public async Task<int> Delete(int id)
{
// Borrar del Api
var response = await _client.DeleteAsync($"{Literals.URI_API_COUNTRIES}/{id}");
if (response.StatusCode == HttpStatusCode.NoContent)
return 1;
else
{
string str = await response.Content.ReadAsStringAsync();
throw new Exception(str);
}
}
}
}
<file_sep>/Countries.Common/Classes/CountryParameters.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Countries.Common.Classes
{
public class CountryParameters : PagingParameters
{
public string Name { get; set; }
public string Alpha2 { get; set; }
public bool OrderByNumericCode { get; set; }
}
}
<file_sep>/Countries.Models/Models/Country.cs
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
using System.ComponentModel.DataAnnotations.Schema;
using System.Text.Json.Serialization;
namespace Countries.Models.Models
{
[Table("Country")]
public class Country
{
[Key]
public int CountryID { get; set; }
[Required(ErrorMessage = "The field {0} is required")]
[StringLength(30)]
public string Name { get; set; }
[StringLength(2)]
public string Alpha_2 { get; set; }
[StringLength(3)]
public string Alpha_3 { get; set; }
[StringLength(3)]
public string NumericCode { get; set; }
public ICollection<Subdivision> Subdivisions { get; set; }
}
}
<file_sep>/Countries.Infra.Data/DataContext/CountriesDbContext.cs
using Countries.Models.Models;
using Microsoft.AspNetCore.Identity.EntityFrameworkCore;
using Microsoft.EntityFrameworkCore;
namespace Countries.Infra.Data.DataContext
{
public class CountriesDbContext : IdentityDbContext
{
public CountriesDbContext(DbContextOptions<CountriesDbContext> options)
: base(options) { }
public DbSet<Country> Countries { get; set; }
public DbSet<Subdivision> Subdivisions { get; set; }
}
}
<file_sep>/Countries.SPA/Services/SubdivisionsHttpService.cs
using Application.DTO;
using Application.Helpers;
using Countries.Common.Classes;
using Countries.Common.Resources;
using Countries.SPA.Helpers.Paging;
using Microsoft.AspNetCore.WebUtilities;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Net.Http;
using System.Net.Http.Json;
using System.Text.Json;
using System.Threading.Tasks;
namespace Countries.SPA.Services
{
public interface ISubdivisionsHttpService
{
Task<PagingResponse<SubdivisionDTO>> GetSubdivisionsDTOAsync(SubdivisionParameter parameters);
Task<SubdivisionDTO> GetByID(int id);
Task<SubdivisionDTO> Create(SubdivisionDTO model);
Task<SubdivisionDTO> Update(SubdivisionDTO model);
Task<int> Delete(int id);
}
public class SubdivisionsHttpService : ISubdivisionsHttpService
{
private readonly HttpClient _client;
public SubdivisionsHttpService(HttpClient client) => this._client = client;
public async Task<PagingResponse<SubdivisionDTO>> GetSubdivisionsDTOAsync(SubdivisionParameter parameters)
{
var queryStringParam = new Dictionary<string, string>
{
["pageNumber"] = parameters.PageNumber.ToString()
};
var response = await _client.GetAsync(QueryHelpers.AddQueryString(Literals.URI_API_SUBDIVISIONS, queryStringParam));
var content = await response.Content.ReadAsStringAsync();
if (!response.IsSuccessStatusCode)
{
throw new ApplicationException(content);
}
var pagingResponse = new PagingResponse<SubdivisionDTO>
{
Items = JsonSerializer.Deserialize<List<SubdivisionDTO>>(content, new JsonSerializerOptions { PropertyNameCaseInsensitive = true }),
MetaData = JsonSerializer.Deserialize<MetaData>(response.Headers.GetValues("X-Pagination").First(), new JsonSerializerOptions { PropertyNameCaseInsensitive = true })
};
return pagingResponse;
}
public async Task<SubdivisionDTO> GetByID(int id)
{
var response = await this._client.GetFromJsonAsync<SubdivisionDTO>($"{Literals.URI_API_SUBDIVISIONS}/{id}");
return response;
}
public async Task<SubdivisionDTO> Create(SubdivisionDTO model)
{
// Insertar en el api
var response = await this._client.PostAsJsonAsync(Literals.URI_API_SUBDIVISIONS, model);
if (response.StatusCode == HttpStatusCode.Created)
{
model = await response.Content.ReadFromJsonAsync<SubdivisionDTO>();
return model;
}
else
{
string str = await response.Content.ReadAsStringAsync();
throw new Exception(str);
}
}
public async Task<SubdivisionDTO> Update(SubdivisionDTO model)
{
var response = await _client.PutAsJsonAsync(Literals.URI_API_SUBDIVISIONS, model);
if (response.StatusCode == HttpStatusCode.OK)
{
model = await response.Content.ReadFromJsonAsync<SubdivisionDTO>();
return model;
}
else
{
string str = await response.Content.ReadAsStringAsync();
throw new Exception(str);
}
}
public async Task<int> Delete(int id)
{
// Borrar del Api
var response = await _client.DeleteAsync($"{Literals.URI_API_SUBDIVISIONS}/{id}");
if (response.StatusCode == HttpStatusCode.NoContent)
return 1;
else
{
string str = await response.Content.ReadAsStringAsync();
throw new Exception(str);
}
}
}
}<file_sep>/Countries.UnitTests/CountriesControllerTests.cs
using Application.Interfaces;
using Countries.API.Controllers;
using Countries.Models.Models;
using Microsoft.AspNetCore.Mvc;
using Moq;
using System.Threading.Tasks;
using Xunit;
namespace Countries.UnitTests
{
public class CountriesControllerTests
{
[Fact]
public async Task Create_ReturnsBadRequest_GivenInvalidModel()
{
// Arrange
var mockRepo = new Mock<ICountriesService>();
var controller = new CountriesController(mockRepo.Object);
controller.ModelState.AddModelError("error", "some error");
// Act
var result = await controller.PostCountry(country: null);
// Assert
Assert.IsType<BadRequestObjectResult>(result.Result);
}
[Fact]
public async Task GetCountry_IsOfType_ActionResult_Country()
{
// Arrange
var mockRepo = new Mock<ICountriesService>();
var controller = new CountriesController(mockRepo.Object);
// Act
var result = await controller.GetCountry(45);
// Assert
Assert.IsType<ActionResult<Country>>(result);
}
}
}
<file_sep>/Countries.API/Controllers/SubdivisionsController.cs
using Application.Interfaces;
using Countries.Common.Classes;
using Countries.Models.Models;
using Microsoft.AspNetCore.Mvc;
using Newtonsoft.Json;
using System.Collections.Generic;
using System.Net;
using System.Threading.Tasks;
namespace Countries.API.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class SubdivisionsController : ControllerBase
{
private readonly ISubdivisionsService _service;
public SubdivisionsController(ISubdivisionsService service) => this._service = service;
// GET: api/Subdivisions
[HttpGet]
public async Task<IEnumerable<Subdivision>> GetCountries([FromQuery] SubdivisionParameter parameters)
{
if (parameters == null)
return null;
var subdivisions = await this._service.GetSubdivisions(parameters);
Response.Headers.Add("X-Pagination", JsonConvert.SerializeObject(subdivisions.MetaData));
return subdivisions;
}
// GET: api/Subdivisions/5
[HttpGet("{SubdivisionID}")]
public async Task<ActionResult<Subdivision>> GetSubdivision(int SubdivisionID)
{
if (SubdivisionID <= 0)
return BadRequest("Invalid ID");
var subdivision = await Task.Run(() => this._service.GetSubdivision(SubdivisionID));
if (subdivision == null)
return NotFound();
return subdivision;
}
// POST: api/Subdivisions
[HttpPost]
public async Task<ActionResult<Subdivision>> PostSubdivision([FromBody] Subdivision subdivision)
{
if (subdivision == null)
return BadRequest("Error when trying to create the country");
await this._service.CreateSubdivisionAsync(subdivision);
return StatusCode((int)HttpStatusCode.Created, subdivision);
}
// PUT: api/Subdivisions
[HttpPut]
public async Task<IActionResult> PutSubdivision([FromBody] Subdivision subdivision)
{
if (subdivision == null)
return BadRequest();
var updated = await this._service.UpdateSubdivisionAsync(subdivision);
return Ok(updated);
}
// DELETE: api/Subdivisions/5
[HttpDelete("{SubdivisionID}")]
public async Task<IActionResult> DeleteSubdivision(int SubdivisionID)
{
if (SubdivisionID <= 0)
return BadRequest("Invalid ID");
var subdivision = this._service.GetSubdivision(SubdivisionID);
if (subdivision == null)
return NotFound();
await this._service.DeleteSubdivisionAsync(subdivision);
return NoContent();
}
}
}<file_sep>/Countries.Infra.Data/Repositories/Generic/BaseRepository.cs
using Microsoft.EntityFrameworkCore;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Linq.Expressions;
using System.Threading.Tasks;
namespace Countries.Infra.Data.Repositories.Generic
{
public interface IBaseRepository<T> where T : class
{
IEnumerable<T> Get(Expression<Func<T, bool>> whereCondition = null,
Func<IQueryable<T>, IOrderedQueryable<T>> orderBy = null,
string includeProperties = "");
Task<T> CreateAsync(T entity);
Task<T> UpdateAsync(T entity, object key);
Task<int> DeleteAsync(T entity);
}
public abstract class BaseRepository<T> : IBaseRepository<T> where T : class
{
private readonly IUnitOfWork _unitOfWork;
public BaseRepository(IUnitOfWork unitOfWork)
{
_unitOfWork = unitOfWork;
}
public IEnumerable<T> Get(Expression<Func<T, bool>> whereCondition = null,
Func<IQueryable<T>, IOrderedQueryable<T>> orderBy = null,
string includeProperties = "")
{
IQueryable<T> query = _unitOfWork.Context.Set<T>();
if (whereCondition != null)
{
query = query.Where(whereCondition);
}
foreach (var includeProperty in includeProperties.Split
(new char[] { ',' }, StringSplitOptions.RemoveEmptyEntries))
{
query = query.Include(includeProperty);
}
if (orderBy != null)
{
return orderBy(query).ToList();
}
else
{
return query.ToList();
}
}
public virtual async Task<T> CreateAsync(T model)
{
_unitOfWork.Context.Set<T>().Add(model);
await _unitOfWork.Context.SaveChangesAsync();
return model;
}
public virtual async Task<T> UpdateAsync(T model, object key)
{
if (model == null)
return null;
T exist = await _unitOfWork.Context.Set<T>().FindAsync(key);
if (exist != null)
{
_unitOfWork.Context.Entry(exist).CurrentValues.SetValues(model);
await _unitOfWork.Context.SaveChangesAsync();
}
return exist;
}
public virtual async Task<int> DeleteAsync(T model)
{
_unitOfWork.Context.Set<T>().Remove(model);
return await _unitOfWork.Context.SaveChangesAsync();
}
}
}
<file_sep>/Application/Services/SubdivisionsService.cs
using Application.Helpers;
using Application.Interfaces;
using Countries.Common.Classes;
using Countries.Infra.Data.Repositories.Custom;
using Countries.Models.Models;
using System;
using System.Collections.Generic;
using System.Threading.Tasks;
namespace Application.Services
{
public class SubdivisionsService : ISubdivisionsService
{
private readonly ISubdivisionsRepository _repository;
public SubdivisionsService(ISubdivisionsRepository repository) => this._repository = repository;
public async Task<PagedList<Subdivision>> GetSubdivisions(SubdivisionParameter parameters)
{
try
{
var result = new List<Subdivision>();
if (parameters != null)
{
result = this._repository.Get(null, null, string.Empty) as List<Subdivision>;
}
return await Task.Run(() => PagedList<Subdivision>.ToPagedList(result, parameters.PageNumber, parameters.PageSize));
}
catch (Exception)
{
throw;
}
}
public async Task<Subdivision> CreateSubdivisionAsync(Subdivision subdivision)
{
Subdivision created = new();
try
{
if (subdivision != null)
{
created = await this._repository.CreateAsync(subdivision);
}
}
catch (Exception)
{
throw;
}
return created;
}
public Subdivision GetSubdivision(int id)
{
Subdivision result = null;
try
{
List<Subdivision> subdivision = this._repository.Get(x => x.SubdivisonID == id, null, string.Empty) as List<Subdivision>;
if (subdivision.Count > 0)
result = subdivision[0];
}
catch (Exception)
{
throw;
}
return result;
}
public async Task<Subdivision> UpdateSubdivisionAsync(Subdivision subdivision)
{
Subdivision updated = new();
try
{
if (subdivision != null)
{
updated = await this._repository.UpdateAsync(subdivision, subdivision.SubdivisonID);
}
}
catch (Exception)
{
throw;
}
return updated;
}
public async Task<int> DeleteSubdivisionAsync(Subdivision subdivision)
{
int deleted = 0;
try
{
if (subdivision != null)
deleted = await this._repository.DeleteAsync(subdivision);
}
catch (Exception)
{
throw;
}
return deleted;
}
}
}
<file_sep>/Countries.Infra.Data/Migrations/20210307181132_Countries_and_Subdivisions_Models.cs
using Microsoft.EntityFrameworkCore.Migrations;
namespace Countries.Infra.Data.Migrations
{
public partial class Countries_and_Subdivisions_Models : Migration
{
protected override void Up(MigrationBuilder migrationBuilder)
{
migrationBuilder.CreateTable(
name: "Country",
columns: table => new
{
CountryID = table.Column<int>(type: "int", nullable: false)
.Annotation("SqlServer:Identity", "1, 1"),
Name = table.Column<string>(type: "nvarchar(30)", maxLength: 30, nullable: false),
Alpha_2 = table.Column<string>(type: "nvarchar(2)", maxLength: 2, nullable: true),
Alpha_3 = table.Column<string>(type: "nvarchar(3)", maxLength: 3, nullable: true),
NumericCode = table.Column<string>(type: "nvarchar(3)", maxLength: 3, nullable: true)
},
constraints: table =>
{
table.PrimaryKey("PK_Country", x => x.CountryID);
});
migrationBuilder.CreateTable(
name: "Subdivison",
columns: table => new
{
SubdivisonID = table.Column<int>(type: "int", nullable: false)
.Annotation("SqlServer:Identity", "1, 1"),
Name = table.Column<string>(type: "nvarchar(30)", maxLength: 30, nullable: false),
CountryID = table.Column<int>(type: "int", nullable: false)
},
constraints: table =>
{
table.PrimaryKey("PK_Subdivison", x => x.SubdivisonID);
table.ForeignKey(
name: "FK_Subdivison_Country_CountryID",
column: x => x.CountryID,
principalTable: "Country",
principalColumn: "CountryID",
onDelete: ReferentialAction.Cascade);
});
migrationBuilder.CreateIndex(
name: "IX_Subdivison_CountryID",
table: "Subdivison",
column: "CountryID");
}
protected override void Down(MigrationBuilder migrationBuilder)
{
migrationBuilder.DropTable(
name: "Subdivison");
migrationBuilder.DropTable(
name: "Country");
}
}
}
<file_sep>/Countries.SPA/Middleware/IoC.cs
using Countries.SPA.Services;
using Countries.SPA.StateProvider;
using Microsoft.AspNetCore.Components.Authorization;
using Microsoft.AspNetCore.Components.WebAssembly.Hosting;
using Microsoft.Extensions.DependencyInjection;
namespace Countries.SPA.Middleware
{
public static class IoC
{
public static WebAssemblyHostBuilder AddDependency(this WebAssemblyHostBuilder builder)
{
// Configuración del AuthorizationStateProvider
builder.Services.AddScoped<JWTAuthStateProvider>();
builder.Services.AddScoped<AuthenticationStateProvider, JWTAuthStateProvider>(provider => provider.GetRequiredService<JWTAuthStateProvider>());
builder.Services.AddScoped<ILoginService, JWTAuthStateProvider>(provider => provider.GetRequiredService<JWTAuthStateProvider>());
// Inject CountryService
builder.Services.AddScoped<ICountriesHttpService, CountriesHttpService>();
builder.Services.AddScoped<ISubdivisionsHttpService, SubdivisionsHttpService>();
return builder;
}
}
}
<file_sep>/Application/Services/JWTService.cs
using Application.Interfaces;
using Countries.Common.Classes;
using Microsoft.AspNetCore.Identity;
using Microsoft.Extensions.Configuration;
using Microsoft.IdentityModel.Tokens;
using System;
using System.Collections.Generic;
using System.IdentityModel.Tokens.Jwt;
using System.Security.Claims;
using System.Text;
using System.Threading.Tasks;
namespace Application.Services
{
public class JWTService : IJWTService
{
private readonly UserManager<IdentityUser> _userManager;
private readonly SignInManager<IdentityUser> _signInManager;
private readonly IConfiguration _configuration;
public JWTService(UserManager<IdentityUser> userManager, SignInManager<IdentityUser> signInManager, IConfiguration configuration)
{
_userManager = userManager;
_signInManager = signInManager;
_configuration = configuration;
}
public async Task<UserToken> BuildToken(UserInfo userInfo)
{
var user = await _signInManager.UserManager.FindByEmailAsync(userInfo.Email);
var roles = await _signInManager.UserManager.GetRolesAsync(user);
var claims = new List<Claim>();
claims.Add(new Claim(JwtRegisteredClaimNames.UniqueName, userInfo.Email));
claims.Add(new Claim(ClaimTypes.Name, user.Email));
claims.Add(new Claim(JwtRegisteredClaimNames.NameId, user.Id));
foreach (var role in roles)
{
claims.Add(new Claim(ClaimTypes.Role, role));
}
var key = new SymmetricSecurityKey(Encoding.UTF8.GetBytes(_configuration["JwtSecurityKey"]));
var creds = new SigningCredentials(key, SecurityAlgorithms.HmacSha256);
// Tiempo de expiración del token. En nuestro caso lo hacemos de una hora.
var expiration = DateTime.UtcNow.AddYears(1);
JwtSecurityToken token = new JwtSecurityToken(
_configuration["JwtIssuer"],
_configuration["JwtAudience"],
claims: claims,
expires: expiration,
signingCredentials: creds);
return new UserToken()
{
Token = new JwtSecurityTokenHandler().WriteToken(token),
Expiration = expiration
};
}
public JwtSecurityToken DecodeJwt(string jwt)
{
var key = Encoding.ASCII.GetBytes(this._configuration.GetSection("JwtSecurityKey").Value);
var handler = new JwtSecurityTokenHandler();
var validations = new TokenValidationParameters
{
ValidateIssuerSigningKey = true,
IssuerSigningKey = new SymmetricSecurityKey(key),
ValidateIssuer = false,
ValidateAudience = false
};
var claims = handler.ValidateToken(jwt, validations, out var tokenSecure);
return (JwtSecurityToken)tokenSecure;
}
}
}
<file_sep>/Application/Interfaces/ICountriesService.cs
using Application.Helpers;
using Countries.Common.Classes;
using Countries.Models.Models;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Application.Interfaces
{
public interface ICountriesService
{
Task<PagedList<Country>> GetCountries(CountryParameters parameters);
Country GetCountry(int id);
Task<Country> CreateCountryAsync(Country country);
Task<Country> UpdateCountryAsync(Country country);
Task<int> DeleteCountryAsync(Country country);
}
}
<file_sep>/Countries.Infra.Data/Repositories/Generic/UnitOfWork.cs
using Countries.Infra.Data.DataContext;
using System;
namespace Countries.Infra.Data.Repositories.Generic
{
public interface IUnitOfWork : IDisposable
{
CountriesDbContext Context { get; }
void Commit();
}
public class UnitOfWork : IUnitOfWork
{
public CountriesDbContext Context { get; }
public UnitOfWork(CountriesDbContext context)
{
Context = context;
}
public void Commit()
{
Context.SaveChanges();
}
public void Dispose()
{
Context.Dispose();
}
}
}
<file_sep>/Countries.Infra.Data/Repositories/Custom/SubdivisionsRepository.cs
using Countries.Infra.Data.Repositories.Generic;
using Countries.Models.Models;
namespace Countries.Infra.Data.Repositories.Custom
{
public interface ISubdivisionsRepository : IBaseRepository<Subdivision>
{
}
public class SubdivisionsRepository : BaseRepository<Subdivision>, ISubdivisionsRepository
{
public SubdivisionsRepository(IUnitOfWork _unitOfWork)
: base(_unitOfWork)
{ }
}
}
<file_sep>/Application/DTO/SubdivisionDTO.cs
using Countries.Models.Models;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Application.DTO
{
public class SubdivisionDTO : Subdivision
{
}
}
<file_sep>/Countries.API/Controllers/CountriesController.cs
using Application.Helpers;
using Application.Interfaces;
using Countries.Common.Classes;
using Countries.Models.Models;
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Mvc;
using Newtonsoft.Json;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Threading.Tasks;
namespace Countries.API.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class CountriesController : ControllerBase
{
private readonly ICountriesService _service;
public CountriesController(ICountriesService service) => this._service = service;
// GET: api/Countries
[HttpGet]
public async Task<IEnumerable<Country>> GetCountries([FromQuery] CountryParameters parameters)
{
if (parameters == null)
return null;
var countries = await this._service.GetCountries(parameters);
Response.Headers.Add("X-Pagination", JsonConvert.SerializeObject(countries.MetaData));
return countries;
}
// GET: api/Countries/5
[HttpGet("{CountryID}")]
public async Task<ActionResult<Country>> GetCountry(int CountryID)
{
if (CountryID <= 0)
return BadRequest("Invalid ID");
var country = await Task.Run(() => this._service.GetCountry(CountryID));
if (country == null)
return NotFound();
return country;
}
// POST: api/Countries
[HttpPost]
public async Task<ActionResult<Country>> PostCountry([FromBody] Country country)
{
if (country == null)
return BadRequest("Error when trying to create the country");
await this._service.CreateCountryAsync(country);
return StatusCode((int)HttpStatusCode.Created, country);
}
// PUT: api/Countries
[HttpPut]
public async Task<IActionResult> PutCountry([FromBody] Country country)
{
if (country == null)
return BadRequest();
var updated = await this._service.UpdateCountryAsync(country);
return Ok(updated);
}
// DELETE: api/Countries/5
[HttpDelete("{CountryID}")]
public async Task<IActionResult> DeleteCountry(int CountryID)
{
if (CountryID <= 0)
return BadRequest("Invalid ID");
var country = this._service.GetCountry(CountryID);
if (country == null)
return NotFound();
await this._service.DeleteCountryAsync(country);
return NoContent();
}
}
}
<file_sep>/Countries.API/Controllers/AccountsController.cs
using Application.Interfaces;
using Countries.Common.Classes;
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Identity;
using Microsoft.AspNetCore.Mvc;
using Microsoft.Extensions.Configuration;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Countries.API.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class AccountsController : ControllerBase
{
private readonly UserManager<IdentityUser> _userManager;
private readonly SignInManager<IdentityUser> _signInManager;
private readonly IConfiguration _configuration;
private readonly IJWTService _jwtService;
public AccountsController(UserManager<IdentityUser> userManager, SignInManager<IdentityUser> signInManager, IConfiguration configuration, IJWTService jwtService)
{
_userManager = userManager;
_signInManager = signInManager;
_configuration = configuration;
_jwtService = jwtService;
}
[HttpPost]
public async Task<ActionResult<UserToken>> Create([FromBody] UserInfo model)
{
var user = new IdentityUser { UserName = model.Email, Email = model.Email };
var result = await _userManager.CreateAsync(user, model.Password);
if (result.Succeeded)
{
// All new users are created with the Admin role
await _userManager.AddToRoleAsync(user, "Admin");
return Ok("Usuario Creado");
}
else
{
StringBuilder errors = new();
foreach (var item in result.Errors)
errors.AppendLine($"{item.Description}");
return BadRequest(errors.ToString());
}
}
[HttpPost("Login")]
public async Task<ActionResult<UserToken>> Login([FromBody] UserInfo userInfo)
{
var result = await _signInManager.PasswordSignInAsync(userInfo.Email, userInfo.Password, isPersistent: false, lockoutOnFailure: false);
if (result.Succeeded)
{
return await this._jwtService.BuildToken(userInfo);
}
else
{
//ModelState.AddModelError("Error", "Intento de inicio de sesión no válido.");
return BadRequest("Intento de inicio de sesión no válido.");
}
}
}
}
| 82bc6899f68816e749a2106f368e0e25ca2360a6 | [
"C#"
] | 29 | C# | EbarriosCode/BackendDotnet_CleanArchitecture | 6be021d568c5b147487a8fe377055e319da3585d | 8859414d78387884ff74f2c5c54cea5840fa550e | |
refs/heads/master | <repo_name>airdispatch/dispatch-blogs<file_sep>/README.md
# Dispatch Blogs
This repository hosts the site of [blogs.airdispat.ch](blogs.airdispat.ch).
<file_sep>/server.go
package main
import (
"airdispat.ch/errors"
"airdispat.ch/identity"
"airdispat.ch/message"
"airdispat.ch/routing"
"airdispat.ch/server"
"airdispat.ch/wire"
"fmt"
"flag"
"getmelange.com/router"
"github.com/airdispatch/go-pressure"
"github.com/russross/blackfriday"
"html/template"
"sort"
"sync"
"time"
)
func main() {
var (
port = flag.Int("port", 0, "port to run the server on")
)
flag.Parse()
portString := fmt.Sprintf(":%d", *port)
s := pressure.CreateServer(portString, true)
id, err := identity.CreateIdentity()
if err != nil {
panic(err)
}
r := &router.Router{
Origin: id,
TrackerList: []string{
"airdispatch.me",
},
Redirects: 10,
}
t := s.CreateTemplateEngine("templates", "base.html")
p := CreatePosts()
viewCounter := CreateViewCount(20)
s.RegisterURL(
// Homepage Route
pressure.NewURLRoute("^/$", &HomepageController{
Templates: t,
Counter: viewCounter,
}),
pressure.NewURLRoute("^/goto$", &GotoController{}),
// Viewer Routes
pressure.NewURLRoute("^/view/(?P<alias>[^@]+@.+?)/(?P<name>.+)$", &ViewPostController{
Templates: t,
Posts: p,
}),
pressure.NewURLRoute("^/view/(?P<alias>[^@]+@.+)$", &ViewerController{
Posts: p,
Templates: t,
Router: r,
Counter: viewCounter,
}),
)
s.RunServer()
}
func errToHTTP(e error) *pressure.HTTPError {
return &pressure.HTTPError{
Code: 500,
Text: e.Error(),
}
}
type HomepageController struct {
Templates *pressure.TemplateEngine
Counter *ViewCount
}
func (c *HomepageController) GetResponse(r *pressure.Request, l *pressure.Logger) (pressure.View, *pressure.HTTPError) {
return c.Templates.NewTemplateView("home.html", map[string]interface{}{
"top": c.Counter.TopAddresses(10),
}), nil
}
type GotoController struct{}
func (c *GotoController) GetResponse(r *pressure.Request, l *pressure.Logger) (pressure.View, *pressure.HTTPError) {
return &RedirectView{
Temporary: true,
Location: fmt.Sprintf("/view/%s", r.Form["address"][0]),
}, nil
}
type RedirectView struct {
pressure.BasicView
Temporary bool
Location string
}
func (r *RedirectView) Headers() pressure.ViewHeaders {
hdrs := r.BasicView.Headers()
if hdrs == nil {
hdrs = make(pressure.ViewHeaders)
}
hdrs["Location"] = r.Location
return hdrs
}
func (r *RedirectView) StatusCode() int {
if r.Temporary {
return 302
}
return 301
}
type blogViews []struct {
Address string
Views int
}
func (c blogViews) Splice(addr string, views int, id int) {
for i := len(c) - 2; i >= id; i -= 1 {
c[i+1] = c[i]
}
c[id] = struct {
Address string
Views int
}{addr, views}
}
func (c blogViews) Len() int { return len(c) }
func (c blogViews) Less(i, j int) bool { return c[i].Views < c[j].Views }
func (c blogViews) Swap(i, j int) { c[i], c[j] = c[j], c[i] }
type ViewCount struct {
lock sync.RWMutex
data map[string]int
tops blogViews
}
func CreateViewCount(n int) *ViewCount {
return &ViewCount{
data: make(map[string]int),
tops: make(blogViews, n),
}
}
func (v *ViewCount) Increment(address string) {
v.lock.Lock()
defer v.lock.Unlock()
viewCount := v.data[address]
viewCount += 1
v.data[address] = viewCount
// Check to see if we are already in the top
for i, blog := range v.tops {
if blog.Address == address {
blog.Views += 1
v.tops[i] = blog
if i != 0 && v.tops[i-1].Views < blog.Views {
v.tops.Swap(i, i-1)
}
return
}
}
outIndex := 0
for i := len(v.tops) - 1; i >= 0; i -= 1 {
outIndex = i
if v.tops[i].Views > viewCount {
break
}
}
if outIndex != len(v.tops)-1 {
v.tops.Splice(address, viewCount, outIndex+1)
}
}
func (v *ViewCount) TopAddresses(num int) blogViews {
v.lock.RLock()
defer v.lock.RUnlock()
if num > len(v.tops) {
panic("Cannot get more top addresses than we are keeping track of.")
}
if num == 0 {
num = len(v.tops)
}
return v.tops[:num]
}
type Collection []*Post
func (c Collection) Len() int { return len(c) }
func (c Collection) Less(i, j int) bool { return c[i].Published.After(c[j].Published) }
func (c Collection) Swap(i, j int) { c[i], c[j] = c[j], c[i] }
type Post struct {
Title string
Body template.HTML
Author string
Name string
Published time.Time
}
type Posts struct {
Data map[string]map[string]*Post
Lock *sync.RWMutex
}
func CreatePosts() *Posts {
return &Posts{
Data: make(map[string]map[string]*Post),
Lock: &sync.RWMutex{},
}
}
func (p *Posts) GetPost(user, id string) *Post {
p.Lock.RLock()
defer p.Lock.RUnlock()
u, ok := p.Data[user]
if !ok {
return nil
}
post, ok := u[id]
if !ok {
return nil
}
return post
}
func (p *Posts) StorePost(user, id string, post *Post) {
p.Lock.Lock()
defer p.Lock.Unlock()
u, ok := p.Data[user]
if !ok {
u = make(map[string]*Post)
}
u[id] = post
p.Data[user] = u
}
type ViewPostController struct {
Templates *pressure.TemplateEngine
Posts *Posts
}
func (v *ViewPostController) GetResponse(r *pressure.Request, l *pressure.Logger) (pressure.View, *pressure.HTTPError) {
alias := r.URL["alias"]
id := r.URL["name"]
post := v.Posts.GetPost(alias, id)
if post == nil {
return nil, &pressure.HTTPError{
Code: 404,
Text: "Could not find that post.",
}
}
return v.Templates.NewTemplateView("viewPost.html", map[string]interface{}{
"user": alias,
"post": post,
}), nil
}
type ViewerController struct {
Templates *pressure.TemplateEngine
Router *router.Router
Posts *Posts
Counter *ViewCount
}
func (v *ViewerController) GetResponse(r *pressure.Request, l *pressure.Logger) (pressure.View, *pressure.HTTPError) {
alias := r.URL["alias"]
srv, err := v.Router.LookupAlias(alias, routing.LookupTypeTX)
if err != nil {
return nil, errToHTTP(err)
}
go v.Counter.Increment(alias)
author, err := v.Router.LookupAlias(alias, routing.LookupTypeMAIL)
if err != nil {
return nil, errToHTTP(err)
}
conn, err := message.ConnectToServer(srv.Location)
if err != nil {
return nil, errToHTTP(err)
}
txMsg := server.CreateTransferMessageList(0, v.Router.Origin.Address, srv, author)
err = message.SignAndSendToConnection(txMsg, v.Router.Origin, srv, conn)
if err != nil {
return nil, errToHTTP(err)
}
recvd, err := message.ReadMessageFromConnection(conn)
if err != nil {
return nil, errToHTTP(err)
}
byt, typ, h, err := recvd.Reconstruct(v.Router.Origin, true)
if err != nil {
return nil, errToHTTP(err)
}
if typ == wire.ErrorCode {
return nil, errToHTTP(
errors.CreateErrorFromBytes(byt, h),
)
} else if typ != wire.MessageListCode {
return nil, errToHTTP(
fmt.Errorf("Expected type (%s), got type (%s)", wire.MessageListCode, typ),
)
}
msgList, err := server.CreateMessageListFromBytes(byt, h)
if err != nil {
return nil, errToHTTP(err)
}
// Get all important messages
var posts Collection
for i := uint64(0); i < msgList.Length; i++ {
newMsg, err := message.ReadMessageFromConnection(conn)
if err != nil {
return nil, errToHTTP(err)
}
byt, typ, h, err := newMsg.Reconstruct(v.Router.Origin, false)
if err != nil {
return nil, errToHTTP(err)
}
if typ == wire.ErrorCode {
return nil, errToHTTP(
errors.CreateErrorFromBytes(byt, h),
)
} else if typ != wire.MailCode {
return nil, errToHTTP(
fmt.Errorf("Expected type (%s), got type (%s)", wire.MailCode, typ),
)
}
// Message is of the correct type.
mail, err := message.CreateMailFromBytes(byt, h)
if err != nil {
return nil, errToHTTP(err)
}
if mail.Components.HasComponent("airdispat.ch/notes/title") {
p := &Post{
Title: mail.Components.GetStringComponent("airdispat.ch/notes/title"),
Body: template.HTML(blackfriday.MarkdownCommon(mail.Components.GetComponent("airdispat.ch/notes/body"))),
Author: alias,
Name: mail.Name,
Published: time.Unix(int64(h.Timestamp), 0),
}
posts = append(posts, p)
v.Posts.StorePost(alias, p.Name, p)
}
}
sort.Sort(posts)
return v.Templates.NewTemplateView("viewer.html", map[string]interface{}{
"user": alias,
"blogs": posts,
}), nil
}
| f806d42fa27acd528c1c0c528a8346c69d1f2053 | [
"Markdown",
"Go"
] | 2 | Markdown | airdispatch/dispatch-blogs | aeba1e3db9ccead96b3a10fcc0e778ea8e78b4f2 | b49b80cbef1e7ded1cb87e8a15530bbec0364839 | |
refs/heads/master | <file_sep>================================================================================
MICROSOFT 基础类库: V9_BATCH_UP 项目概述
===============================================================================
应用程序向导已为您创建了这个 V9_BATCH_UP 应用程序。此应用程序不仅演示 Microsoft 基础类的基本使用方法,还可作为您编写应用程序的起点。
本文件概要介绍组成 V9_BATCH_UP 应用程序的每个文件的内容。
V9_BATCH_UP.vcproj
这是使用应用程序向导生成的 VC++ 项目的主项目文件。
它包含生成该文件的 Visual C++ 的版本信息,以及有关使用应用程序向导选择的平台、配置和项目功能的信息。
V9_BATCH_UP.h
这是应用程序的主要头文件。它包括其他项目特定的头文件(包括 Resource.h),并声明 CV9_BATCH_UPApp 应用程序类。
V9_BATCH_UP.cpp
这是包含应用程序类 CV9_BATCH_UPApp 的主要应用程序源文件。
V9_BATCH_UP.rc
这是程序使用的所有 Microsoft Windows 资源的列表。它包括 RES 子目录中存储的图标、位图和光标。此文件可以直接在 Microsoft Visual C++ 中进行编辑。项目资源位于 2052 中。
res\V9_BATCH_UP.ico
这是用作应用程序图标的图标文件。此图标包括在主要资源文件 V9_BATCH_UP.rc 中。
res\V9_BATCH_UP.rc2
此文件包含不在 Microsoft Visual C++ 中进行编辑的资源。您应该将不可由资源编辑器编辑的所有资源放在此文件中。
/////////////////////////////////////////////////////////////////////////////
应用程序向导创建一个对话框类:
V9_BATCH_UPDlg.h,V9_BATCH_UPDlg.cpp - 对话框
这些文件包含 CV9_BATCH_UPDlg 类。该类定义应用程序主对话框的行为。该对话框的模板位于 V9_BATCH_UP.rc 中,该文件可以在 Microsoft Visual C++ 中进行编辑。
/////////////////////////////////////////////////////////////////////////////
其他功能:
ActiveX 控件
应用程序包括对使用 ActiveX 控件的支持。
/////////////////////////////////////////////////////////////////////////////
其他标准文件:
StdAfx.h,StdAfx.cpp
这些文件用于生成名为 V9_BATCH_UP.pch 的预编译头 (PCH) 文件和名为 StdAfx.obj 的预编译类型文件。
Resource.h
这是标准头文件,它定义新的资源 ID。
Microsoft Visual C++ 读取并更新此文件。
V9_BATCH_UP.manifest
应用程序清单文件供 Windows XP 用来描述应用程序
对特定版本并行程序集的依赖性。加载程序使用此
信息从程序集缓存加载适当的程序集或
从应用程序加载私有信息。应用程序清单可能为了重新分发而作为
与应用程序可执行文件安装在相同文件夹中的外部 .manifest 文件包括,
也可能以资源的形式包括在该可执行文件中。
/////////////////////////////////////////////////////////////////////////////
其他注释:
应用程序向导使用“TODO:”指示应添加或自定义的源代码部分。
如果应用程序在共享的 DLL 中使用 MFC,则需要重新发布这些 MFC DLL;如果应用程序所用的语言与操作系统的当前区域设置不同,则还需要重新发布对应的本地化资源 MFC90XXX.DLL。有关这两个主题的更多信息,请参见 MSDN 文档中有关 Redistributing Visual C++ applications (重新发布 Visual C++ 应用程序)的章节。
/////////////////////////////////////////////////////////////////////////////
<file_sep>//{{NO_DEPENDENCIES}}
// Microsoft Visual C++ generated include file.
// Used by V9_BATCH_UP.rc
//
#define IDM_ABOUTBOX 0x0010
#define IDD_ABOUTBOX 100
#define IDS_ABOUTBOX 101
#define IDD_V9_BATCH_UP_DIALOG 102
#define IDR_MAINFRAME 128
#define IDC_LIST1 1000
#define IDC_LIST2 1001
#define IDC_BUTTON1 1002
#define IDC_BUTTON2 1003
#define IDC_PROGRESS1 1004
#define IDC_RADIO1 1005
#define IDC_RADIO2 1006
#define IDC_EDIT1 1007
#define IDC_BUTTON3 1008
#define IDC_EDIT2 1013
#define IDC_STATIC1 1014
#define IDC_STATIC2 1015
#define IDC_STATIC3 1016
#define IDC_STATIC4 1017
#define IDC_STATIC5 1018
#define IDC_STATIC11 1019
#define IDC_STATIC15 1020
// Next default values for new objects
//
#ifdef APSTUDIO_INVOKED
#ifndef APSTUDIO_READONLY_SYMBOLS
#define _APS_NEXT_RESOURCE_VALUE 132
#define _APS_NEXT_COMMAND_VALUE 32771
#define _APS_NEXT_CONTROL_VALUE 1021
#define _APS_NEXT_SYMED_VALUE 101
#endif
#endif
<file_sep>CC = arm-hisiv200-linux-gcc
OPTIMIZER = -O3
CFLAGS =$(OPTIMIZER) -Wall -D_GNU_SOURCE
OBJS = wibox_batch_up.o
PGM = wibox_batch_up
all:$(PGM)
$(PGM): $(OBJS)
$(CC) -o $(PGM) $(OBJS) -lpthread
clean:
-rm -f *.o $(PGM)
<file_sep>// V9_BATCH_UPDlg.cpp : 实现文件
//
#include "stdafx.h"
#include "V9_BATCH_UP.h"
#include "V9_BATCH_UPDlg.h"
#include <Winsock2.h>
#include <stdio.h>
#include <process.h>
#ifdef _DEBUG
#define new DEBUG_NEW
#endif
static CV9_BATCH_UPDlg *pMain;
UINT __stdcall GetDevThread(void* lpParam) ;
UINT __stdcall updatemain(void* lpParam) ;
UINT __stdcall tcp_client(void* lpParam) ;
#define BATCH_UP_PORT 20000
#define BATCH_UP_PORT_C 20001
#define BATCH_STATUS_PORT 20002
//#define UPDATEDIR "updates\\910"
#define UPDATEDIR ".\\"
#define UPDATENAME "updates"
#define BUFFSIZE 1024
int file_md5(const char *file, char *md5);
int tar_gzip(const char *files, const char *tgz);
unsigned long long file_len(const char *file);
int send_pack(SOCKET s, const char *buf, int len, int flags);
BOOL CreateCSV (char *file);
BOOL AddToCSV (char *file, struct DEVINFOLIST *host, int *status);
HANDLE g_hThreadParameter;
HANDLE g_status[BOARD_MAX];
HANDLE g_status_check[BOARD_MAX];
char md5[50] = {0};
unsigned long long g_file_len;
UINT __stdcall GetDevThread(void* lpParam)
{
CString str;
DEVINFO devinfo;
SOCKET sockSrv = socket(AF_INET, SOCK_DGRAM, 0);
if (sockSrv == INVALID_SOCKET)
{
str.Format ("%s", "socket error");
AfxMessageBox(str);
return -1;
}
SOCKADDR_IN addrSrv;
memset(&addrSrv, 0, sizeof(SOCKADDR_IN));
addrSrv.sin_addr.S_un.S_addr = htonl(INADDR_ANY); // 将INADDR_ANY转换为网络字节序,调用 htonl(long型)或htons(整型)
addrSrv.sin_family = AF_INET;
addrSrv.sin_port = htons(BATCH_UP_PORT);
if(bind(sockSrv, (SOCKADDR*)&addrSrv, sizeof(SOCKADDR)) == SOCKET_ERROR)
{
str.Format ("%s", "bind error");
AfxMessageBox(str);
return -1;
}
//if(listen(sockSrv, 30) == SOCKET_ERROR)
//{
// str.Format ("%s", "listen error");
// AfxMessageBox(str);
// return -1;
//}
SOCKADDR_IN addrClient;
int addrClientsz;
int len=sizeof(SOCKADDR);
char ip[100];
//char buf[100];
while (1)
{
//SOCKET sockConn = recvfrom(sockSrv, (SOCKADDR*)&addrClient, &len);
addrClientsz = sizeof(SOCKADDR_IN);
recvfrom(sockSrv, (char *)&devinfo, sizeof(devinfo), 0, (SOCKADDR *)&addrClient, &addrClientsz);
//sprintf(buf, "client %s connect to the server\n", inet_ntoa(addrClient.sin_addr));
//AfxMessageBox(buf);
sprintf(ip, "%s", inet_ntoa(addrClient.sin_addr));
//recv(sockConn, (char *)&devinfo.type, sizeof(devinfo.type), 0);
devinfo.type = ntohl(devinfo.type);
//sprintf(buf, "%x", ntohl(devinfo.type));
//AfxMessageBox(buf);
//recv(sockConn, devinfo.routeV, sizeof(devinfo.routeV), 0);
//AfxMessageBox(devinfo.routeV);
//recv(sockConn, devinfo.kernelV, sizeof(devinfo.kernelV), 0);
//recv(sockConn, devinfo.appV, sizeof(devinfo.appV), 0);
//recv(sockConn, devinfo.recordV, sizeof(devinfo.recordV), 0);
//AfxMessageBox(devinfo.recordV);
//recv(sockConn, devinfo.bsV, sizeof(devinfo.bsV), 0);
pMain->AddHost(ip, &devinfo);
//pMain->listboard();
//closesocket(sockConn);
}
closesocket(sockSrv);
//WSACleanup();
return 0;
}
UINT __stdcall GetStatusThread(void* lpParam)
{
CString str;
SOCKET sockSrv = socket(AF_INET, SOCK_STREAM, 0);
if (sockSrv == INVALID_SOCKET)
{
str.Format ("%s", "socket error");
AfxMessageBox(str);
return -1;
}
SOCKADDR_IN addrSrv;
memset(&addrSrv, 0, sizeof(SOCKADDR_IN));
addrSrv.sin_addr.S_un.S_addr = htonl(INADDR_ANY); // 将INADDR_ANY转换为网络字节序,调用 htonl(long型)或htons(整型)
addrSrv.sin_family = AF_INET;
addrSrv.sin_port = htons(BATCH_STATUS_PORT);
if(bind(sockSrv, (SOCKADDR*)&addrSrv, sizeof(SOCKADDR)) == SOCKET_ERROR)
{
str.Format ("%s", "bind error");
AfxMessageBox(str);
return -1;
}
if(listen(sockSrv, 30) == SOCKET_ERROR)
{
str.Format ("%s", "listen error");
AfxMessageBox(str);
return -1;
}
SOCKADDR_IN addrClient;
int len=sizeof(SOCKADDR);
char ip[100];
char buf[10];
int sta;
while (1)
{
SOCKET sockConn = accept(sockSrv, (SOCKADDR*)&addrClient, &len);
//sprintf(buf, "client %s connect to the server\n", inet_ntoa(addrClient.sin_addr));
//AfxMessageBox(buf);
sprintf(ip, "%s", inet_ntoa(addrClient.sin_addr));
memset(buf, 0, sizeof(buf));
recv(sockConn, buf, sizeof(buf), 0);
if(strncmp(buf, "status:", 7) != 0)
{
//closesocket(sockSrv);
AfxMessageBox("板卡返回数据有误");
closesocket(sockConn);
continue;
}
if(strlen(buf) < 9)
{
//closesocket(sockSrv);
AfxMessageBox("板卡返回数据有误");
closesocket(sockConn);
continue;
}
sta = atoi(buf+7);
pMain->up_status(ip, sta);
closesocket(sockConn);
}
closesocket(sockSrv);
return 0;
}
UINT __stdcall tcp_client(void* lpParam)
{
int num = *(int *)lpParam;
ReleaseSemaphore(g_hThreadParameter, 1, NULL);//信号量++
//int index = 0;
//char buf[100] = {0};
//sprintf(buf, "%d", num);
//AfxMessageBox(buf);
pMain->tcp_send(num);
return 0;
}
int CV9_BATCH_UPDlg::updatefunc()
{
if(BST_CHECKED==((CButton*)GetDlgItem(IDC_RADIO1))->GetCheck())
{
tcp_update();
}
else if(BST_CHECKED==((CButton*)GetDlgItem(IDC_RADIO2))->GetCheck())
{
ftp_update();
}
((CButton*)GetDlgItem(IDOK))->EnableWindow(TRUE);
((CButton*)GetDlgItem(IDC_BUTTON1))->EnableWindow(TRUE);
((CButton*)GetDlgItem(IDC_BUTTON2))->EnableWindow(TRUE);
return 0;
}
UINT __stdcall updatemain(void* lpParam)
{
//while (pMain->updateflag)
{
pMain->updatefunc();
}
return 0;
}
int CV9_BATCH_UPDlg::insertHost(DEVINFOLIST *pos, const char *ip, struct DEVINFO *info)
{
struct DEVINFOLIST * p = (struct DEVINFOLIST *) malloc (sizeof (DEVINFOLIST));
if (p == NULL)
{
AfxMessageBox("内存不足");
}
memset(p, 0, sizeof(DEVINFOLIST));
strcpy(p->ip, ip);
strcpy(p->name, info->name);
p->type = info->type;
strcpy(p->routeV, info->routeV);
strcpy(p->kernelV, info->kernelV);
strcpy(p->appV, info->appV);
strcpy(p->recordV, info->recordV);
strcpy(p->bsV, info->bsV);
p->pNext = pos->pNext;
pos->pNext = p;
return 0;
}
int CV9_BATCH_UPDlg::insertUpdateHost(DEVINFOLIST *pos, DEVINFOLIST *info)
{
struct DEVINFOLIST * p = (struct DEVINFOLIST *) malloc (sizeof (DEVINFOLIST));
if (p == NULL)
{
AfxMessageBox("内存不足");
}
memcpy(p, info, sizeof(DEVINFOLIST));
p->pNext = pos->pNext;
pos->pNext = p;
return 0;
}
int CV9_BATCH_UPDlg::delUpdateHost(DEVINFOLIST *pre, DEVINFOLIST *pos)
{
pre->pNext = pos->pNext;
free(pos);
return 0;
}
int CV9_BATCH_UPDlg::listboard()
{
DEVINFOLIST * p = m_pHost->pNext;
CString str;
int index = 0;
m_listboard.DeleteAllItems();
while(p != NULL)
{
str.Format ("%d", index+1);
m_listboard.InsertItem(index, str);
str.Format ("%s", p->ip);
m_listboard.SetItemText(index, 1, str);
str.Format ("%s", p->name);
m_listboard.SetItemText(index, 2, str);
str.Format ("0x%x", p->type);
m_listboard.SetItemText(index, 3, str);
str.Format ("%s", p->routeV);
m_listboard.SetItemText(index, 4, str);
str.Format ("%s", p->kernelV);
m_listboard.SetItemText(index, 5, str);
str.Format ("%s", p->appV);
m_listboard.SetItemText(index, 6, str);
str.Format ("%s", p->recordV);
m_listboard.SetItemText(index, 7, str);
str.Format ("%s", p->bsV);
m_listboard.SetItemText(index, 8, str);
p = p->pNext;
index++;
}
char buf[10] = {0};
sprintf(buf, "%d", index);
GetDlgItem(IDC_STATIC15)->SetWindowText(buf);
return 0;
}
int CV9_BATCH_UPDlg::listUpdateboard()
{
DEVINFOLIST * p = m_pHostUpdate->pNext;
CString str;
int index = 0;
m_updateboard.DeleteAllItems();
while(p != NULL)
{
str.Format ("No.%d.%s@IP:%s type:0x%x", index+1, p->name, p->ip, p->type);
m_updateboard.InsertItem(index, str);
p = p->pNext;
index++;
}
return 0;
}
BOOL CV9_BATCH_UPDlg::up_status(const char *ip, int sta)
{
DEVINFOLIST * p = m_pHostUpdate->pNext;
CString str;
int index = 0;
while(p != NULL)
{
if(strcmp(p->ip, ip) == 0)
{
if(status[index] != sta)
{
status[index] = sta;
update_rec_flag(sta, p->ip, index);
if(sta != UP_TRANS_SUC && sta != UP_MD5_SUC)
ReleaseSemaphore(g_status[index], 1, NULL);//信号量++
break;
}
}
p = p->pNext;
index++;
}
return true;
}
BOOL CV9_BATCH_UPDlg::AddHost(const char *ip, struct DEVINFO *info)
{
CString str;
struct DEVINFOLIST * p = m_pHost->pNext;
struct DEVINFOLIST * pPre = m_pHost;
int change_flag = 0;
if (p == NULL)
{
//AfxMessageBox("NULL");
insertHost(m_pHost, ip, info);
listboard();
}
else
{
//AfxMessageBox("not NULL");
while (p != NULL)
{
//AfxMessageBox(ip);
//AfxMessageBox(p->ip);
if(strcmp(p->ip, ip) == 0)
{
if(strcmp(p->name, info->name) != 0)
{
change_flag = 1;
strcpy(p->name, info->name);
}
if(strcmp(p->routeV, info->routeV) != 0)
{
change_flag = 1;
strcpy(p->routeV, info->routeV);
}
if(strcmp(p->kernelV, info->kernelV) != 0)
{
change_flag = 1;
strcpy(p->kernelV, info->kernelV);
}
if(strcmp(p->appV, info->appV) != 0)
{
change_flag = 1;
strcpy(p->appV, info->appV);
}
if(strcmp(p->recordV, info->recordV) != 0)
{
change_flag = 1;
strcpy(p->recordV, info->recordV);
}
if(strcmp(p->bsV, info->bsV) != 0)
{
change_flag = 1;
strcpy(p->bsV, info->bsV);
}
if(p->type != info->type)
{
change_flag = 1;
p->type = info->type;
}
if(change_flag == 1)
listboard();
return FALSE;
}
else if(strcmp(p->ip, ip) > 0)
{
insertHost(pPre, ip, info);
listboard();
return TRUE;
}
else
{
pPre = p;
p = p->pNext;
}
}
insertHost(pPre, ip, info);
listboard();
}
return TRUE;
}
BOOL CV9_BATCH_UPDlg::AddBoardUpdate (int nIndex)
{
DEVINFOLIST * pPre = m_pHostUpdate;
DEVINFOLIST * pHost = m_pHost->pNext;
DEVINFOLIST *pUpdate = m_pHostUpdate->pNext;
for (int i = 0; i < nIndex; i++)
{
pHost = pHost->pNext;
}
if (pUpdate== NULL)
{
insertUpdateHost(m_pHostUpdate, pHost);
//listUpdateboard();
}
else
{
while (pUpdate != NULL)
{
if (strcmp(pUpdate->ip, pHost->ip) == 0)
{
return FALSE;
}
else if(strcmp(pUpdate->ip, pHost->ip) > 0)
{
insertUpdateHost(pPre, pHost);
//listUpdateboard();
return TRUE;
}
else
{
pPre = pUpdate;
pUpdate = pUpdate->pNext;
}
}
insertUpdateHost(pPre, pHost);
//listUpdateboard();
}
return TRUE;
}
BOOL CV9_BATCH_UPDlg::DelBoardUpdate (const char *ip)
{
DEVINFOLIST * pPre = m_pHostUpdate;
DEVINFOLIST * pHost = m_pHostUpdate->pNext;
DEVINFOLIST *pUpdate = m_pHostUpdate->pNext;
if(pHost == NULL)
{
AfxMessageBox("NULL");
return FALSE;
}
while(pHost != NULL)
{
if(strcmp(ip, pHost->ip) == 0)
{
break;
}
pPre = pHost;
pHost = pHost->pNext;
}
delUpdateHost(pPre, pHost);
return TRUE;
}
int CV9_BATCH_UPDlg::FindUpdates()
{
WIN32_FIND_DATA FindData;
int ret;
char *psta,*pend;
char namebuf[512] = UPDATEDIR"*.tar";
char version[100] = {0};
int have_file = 0;
HANDLE hFind = FindFirstFile(namebuf, &FindData );
if(hFind == INVALID_HANDLE_VALUE)
{
AfxMessageBox("当前升级目录不存在任何升级包");
return -1;
}
while (1)
{
//AfxMessageBox(FindData.cFileName);
if (strncmp(FindData.cFileName, "route", 5) == 0)
{
//AfxMessageBox("route");
strcpy(updatefile.route, FindData.cFileName);
psta = updatefile.route + 5;
pend = strstr(updatefile.route, ".tar");
if (pend == NULL)
{
AfxMessageBox("升级文件名有误");
memset(updatefile.route, 0, sizeof(updatefile.route));
return -2;
}
memset(version, 0, sizeof(version));
memcpy(version, psta+1, pend-psta-1);
GetDlgItem(IDC_STATIC1)->SetWindowText(version);
have_file = 1;
}
if (strncmp(FindData.cFileName, "kernel", 6) == 0)
{
//AfxMessageBox("kernel");
strcpy(updatefile.kernel, FindData.cFileName);
psta = updatefile.kernel + 6;
pend = strstr(updatefile.kernel, ".tar");
if (pend == NULL)
{
AfxMessageBox("升级文件名有误");
memset(updatefile.kernel, 0, sizeof(updatefile.kernel));
return -2;
}
memset(version, 0, sizeof(version));
memcpy(version, psta+1, pend-psta-1);
GetDlgItem(IDC_STATIC2)->SetWindowText(version);
have_file = 1;
}
if (strncmp(FindData.cFileName, "app", 3) == 0)
{
//AfxMessageBox("v9-wibox");
strcpy(updatefile.app, FindData.cFileName);
psta = updatefile.app + 3;
pend = strstr(updatefile.app, ".tar");
if (pend == NULL)
{
AfxMessageBox("升级文件名有误");
memset(updatefile.app, 0, sizeof(updatefile.app));
return -2;
}
memset(version, 0, sizeof(version));
memcpy(version, psta+1, pend-psta-1);
GetDlgItem(IDC_STATIC3)->SetWindowText(version);
have_file = 1;
}
if (strncmp(FindData.cFileName, "record", 6) == 0)
{
//AfxMessageBox("record");
strcpy(updatefile.record, FindData.cFileName);
psta = updatefile.record + 6;
pend = strstr(updatefile.record, ".tar");
if (pend == NULL)
{
AfxMessageBox("升级文件名有误");
memset(updatefile.record, 0, sizeof(updatefile.record));
return -2;
}
memset(version, 0, sizeof(version));
memcpy(version, psta+1, pend-psta-1);
GetDlgItem(IDC_STATIC4)->SetWindowText(version);
have_file = 1;
}
if (strncmp(FindData.cFileName, "bs", 2) == 0)
{
//AfxMessageBox("bs");
strcpy(updatefile.bs, FindData.cFileName);
psta = updatefile.bs + 2;
pend = strstr(updatefile.bs, ".tar");
if (pend == NULL)
{
AfxMessageBox("升级文件名有误");
memset(updatefile.bs, 0, sizeof(updatefile.bs));
return -2;
}
memset(version, 0, sizeof(version));
memcpy(version, psta+1, pend-psta-1);
GetDlgItem(IDC_STATIC5)->SetWindowText(version);
have_file = 1;
}
ret = FindNextFile(hFind,&FindData);
if (!ret)
{
//AfxMessageBox("FindNextFile error");
break;
}
}
FindClose(hFind);
if(have_file == 0)
{
AfxMessageBox("当前目录不存在任何升级文件,请确认整备完成后,重新打开进行升级");
return -5;
}
return 0;
}
// 用于应用程序“关于”菜单项的 CAboutDlg 对话框
class CAboutDlg : public CDialog
{
public:
CAboutDlg();
// 对话框数据
enum { IDD = IDD_ABOUTBOX };
protected:
virtual void DoDataExchange(CDataExchange* pDX); // DDX/DDV 支持
// 实现
protected:
DECLARE_MESSAGE_MAP()
};
CAboutDlg::CAboutDlg() : CDialog(CAboutDlg::IDD)
{
}
void CAboutDlg::DoDataExchange(CDataExchange* pDX)
{
CDialog::DoDataExchange(pDX);
}
BEGIN_MESSAGE_MAP(CAboutDlg, CDialog)
END_MESSAGE_MAP()
// CV9_BATCH_UPDlg 对话框
CV9_BATCH_UPDlg::CV9_BATCH_UPDlg(CWnd* pParent /*=NULL*/)
: CDialog(CV9_BATCH_UPDlg::IDD, pParent)
{
m_hIcon = AfxGetApp()->LoadIcon(IDR_MAINFRAME);
}
void CV9_BATCH_UPDlg::DoDataExchange(CDataExchange* pDX)
{
CDialog::DoDataExchange(pDX);
DDX_Control(pDX, IDC_LIST2, m_listboard);
DDX_Control(pDX, IDC_LIST1, m_updateboard);
}
BEGIN_MESSAGE_MAP(CV9_BATCH_UPDlg, CDialog)
ON_WM_SYSCOMMAND()
ON_WM_PAINT()
ON_WM_QUERYDRAGICON()
//}}AFX_MSG_MAP
ON_BN_CLICKED(IDC_RADIO1, &CV9_BATCH_UPDlg::OnBnClickedRadio1)
ON_BN_CLICKED(IDC_RADIO2, &CV9_BATCH_UPDlg::OnBnClickedRadio2)
ON_BN_CLICKED(IDC_BUTTON1, &CV9_BATCH_UPDlg::OnBnClickedButton1)
ON_BN_CLICKED(IDC_BUTTON2, &CV9_BATCH_UPDlg::OnBnClickedButton2)
ON_BN_CLICKED(IDC_BUTTON3, &CV9_BATCH_UPDlg::OnBnClickedButton3)
ON_BN_CLICKED(IDOK, &CV9_BATCH_UPDlg::OnBnClickedOk)
END_MESSAGE_MAP()
// CV9_BATCH_UPDlg 消息处理程序
BOOL CV9_BATCH_UPDlg::OnInitDialog()
{
CDialog::OnInitDialog();
// 将“关于...”菜单项添加到系统菜单中。
pMain = this;
m_pHost = (struct DEVINFOLIST *) malloc (sizeof (DEVINFOLIST));
memset(m_pHost, 0, sizeof(DEVINFOLIST));
m_pHost->pNext = NULL;
m_pHostUpdate = (struct DEVINFOLIST *) malloc (sizeof (DEVINFOLIST));
memset(m_pHostUpdate, 0, sizeof(DEVINFOLIST));
m_pHostUpdate->pNext = NULL;
memset(&updatefile, 0, sizeof(updatefile));
// IDM_ABOUTBOX 必须在系统命令范围内。
ASSERT((IDM_ABOUTBOX & 0xFFF0) == IDM_ABOUTBOX);
ASSERT(IDM_ABOUTBOX < 0xF000);
CMenu* pSysMenu = GetSystemMenu(FALSE);
if (pSysMenu != NULL)
{
CString strAboutMenu;
strAboutMenu.LoadString(IDS_ABOUTBOX);
if (!strAboutMenu.IsEmpty())
{
pSysMenu->AppendMenu(MF_SEPARATOR);
pSysMenu->AppendMenu(MF_STRING, IDM_ABOUTBOX, strAboutMenu);
}
}
// 设置此对话框的图标。当应用程序主窗口不是对话框时,框架将自动
// 执行此操作
SetIcon(m_hIcon, TRUE); // 设置大图标
SetIcon(m_hIcon, FALSE); // 设置小图标
// TODO: 在此添加额外的初始化代码
m_listboard.InsertColumn (0, "ID", LVCFMT_CENTER, 40);
m_listboard.InsertColumn (1, "IP Address", LVCFMT_CENTER, 110);
m_listboard.InsertColumn (2, "Board Name", LVCFMT_CENTER, 80);
m_listboard.InsertColumn (3, "Type", LVCFMT_CENTER, 50);
m_listboard.InsertColumn (4, "route Version", LVCFMT_CENTER, 100);
m_listboard.InsertColumn (5, "kernel Version", LVCFMT_CENTER, 100);
m_listboard.InsertColumn (6, "app Version", LVCFMT_CENTER, 100);
m_listboard.InsertColumn (7, "record Version", LVCFMT_CENTER, 100);
m_listboard.InsertColumn (8, "bs Version", LVCFMT_CENTER, 100);
m_listboard.SetExtendedStyle(
LVS_EX_FULLROWSELECT |
LVS_EX_GRIDLINES | \
LVS_EX_HEADERDRAGDROP);
((CButton*)GetDlgItem(IDC_RADIO1))->SetCheck(TRUE);
((CButton*)GetDlgItem(IDC_EDIT1))->EnableWindow(FALSE);
int ret;
ret = FindUpdates();
if(ret == -2 || ret == -5)
{
//return FALSE;
}
WSADATA wsaData; // 这结构是用于接收Windows Socket的结构信息的
int err;
CString str;
err = WSAStartup( MAKEWORD( 2, 2 ), &wsaData );
if ( err != 0 )
{
str.Format ("%s", "WSAStartup error");
AfxMessageBox(str);
return -1;
}
HANDLE hThread = (HANDLE)_beginthreadex(NULL, 0, GetDevThread, NULL, 0, NULL);
CloseHandle( hThread );
hThread = (HANDLE)_beginthreadex(NULL, 0, GetStatusThread, NULL, 0, NULL);
CloseHandle( hThread );
return TRUE; // 除非将焦点设置到控件,否则返回 TRUE
}
void CV9_BATCH_UPDlg::OnSysCommand(UINT nID, LPARAM lParam)
{
if ((nID & 0xFFF0) == IDM_ABOUTBOX)
{
CAboutDlg dlgAbout;
dlgAbout.DoModal();
}
else
{
CDialog::OnSysCommand(nID, lParam);
}
}
// 如果向对话框添加最小化按钮,则需要下面的代码
// 来绘制该图标。对于使用文档/视图模型的 MFC 应用程序,
// 这将由框架自动完成。
void CV9_BATCH_UPDlg::OnPaint()
{
if (IsIconic())
{
CPaintDC dc(this); // 用于绘制的设备上下文
SendMessage(WM_ICONERASEBKGND, reinterpret_cast<WPARAM>(dc.GetSafeHdc()), 0);
// 使图标在工作区矩形中居中
int cxIcon = GetSystemMetrics(SM_CXICON);
int cyIcon = GetSystemMetrics(SM_CYICON);
CRect rect;
GetClientRect(&rect);
int x = (rect.Width() - cxIcon + 1) / 2;
int y = (rect.Height() - cyIcon + 1) / 2;
// 绘制图标
dc.DrawIcon(x, y, m_hIcon);
}
else
{
CDialog::OnPaint();
}
}
//当用户拖动最小化窗口时系统调用此函数取得光标
//显示。
HCURSOR CV9_BATCH_UPDlg::OnQueryDragIcon()
{
return static_cast<HCURSOR>(m_hIcon);
}
void CV9_BATCH_UPDlg::OnBnClickedRadio1()
{
// TODO: 在此添加控件通知处理程序代码
((CButton*)GetDlgItem(IDC_EDIT2))->EnableWindow(TRUE);
((CButton*)GetDlgItem(IDC_EDIT1))->EnableWindow(FALSE);
}
void CV9_BATCH_UPDlg::OnBnClickedRadio2()
{
// TODO: 在此添加控件通知处理程序代码
((CButton*)GetDlgItem(IDC_EDIT1))->EnableWindow(TRUE);
((CButton*)GetDlgItem(IDC_EDIT2))->EnableWindow(FALSE);
}
void CV9_BATCH_UPDlg::OnBnClickedButton1()
{
// TODO: 在此添加控件通知处理程序代码
CString str;
POSITION pos = m_listboard.GetFirstSelectedItemPosition();
if (pos == NULL)
{
AfxMessageBox(TEXT("没有选中任何板卡"));
}
else
{
while (pos)
{
int nItem = m_listboard.GetNextSelectedItem(pos);
str = m_listboard.GetItemText (nItem, 0);
AddBoardUpdate (atoi (str.GetBuffer ()) - 1);
}
listUpdateboard();
}
}
void CV9_BATCH_UPDlg::OnBnClickedButton2()
{
// TODO: 在此添加控件通知处理程序代码
CString str;
char buf[100] = {0};
POSITION pos = m_updateboard.GetFirstSelectedItemPosition();
if (pos == NULL)
{
AfxMessageBox(TEXT("没有选中任何板卡"));
}
else
{
while (pos)
{
int nItem = m_updateboard.GetNextSelectedItem(pos);
str = m_updateboard.GetItemText (nItem, 0);
//AfxMessageBox(str);
char *p = strstr(str.GetBuffer (), "IP:");
strcpy(buf, p+3);
p = buf;
while(*p != ' ')
{
p++;
}
*p = '\0';
//AfxMessageBox(buf);
DelBoardUpdate (buf);
}
listUpdateboard();
}
}
void CV9_BATCH_UPDlg::OnBnClickedButton3()
{
// TODO: 在此添加控件通知处理程序代码
}
int CV9_BATCH_UPDlg::CProgressCtrlInit(int range)
{
CProgressCtrl *pProgCtrl=(CProgressCtrl*)GetDlgItem(IDC_PROGRESS1);
pProgCtrl->SetRange(0,range);
pProgCtrl->SetStep(1);
pProgCtrl->SetPos(0);
return 0;
}
int CV9_BATCH_UPDlg::CProgressCtrlUpdate(int num, const char *info)
{
CProgressCtrl *pProgCtrl=(CProgressCtrl*)GetDlgItem(IDC_PROGRESS1);
for (int i=0;i<num;i++)
{
pProgCtrl->StepIt();
}
GetDlgItem(IDC_STATIC11)->SetWindowText(info);
Sleep(500);
return 0;
}
int CV9_BATCH_UPDlg::addfile(char *files, const char *file)
{
if(file)
{
if(strlen(file) != 0)
{
strcat(files, UPDATEDIR);
strcat(files, file);
strcat(files, " ");
}
}
return 0;
}
int CV9_BATCH_UPDlg::tar_gz()
{
CProgressCtrlUpdate(1, "打包压缩升级文件中...");
char files[1000] = {0};
addfile(files, updatefile.route);
addfile(files, updatefile.kernel);
addfile(files, updatefile.app);
addfile(files, updatefile.record);
addfile(files, updatefile.bs);
//AfxMessageBox(files);
if(tar_gzip(files, UPDATENAME))
{
return -1;
}
return 0;
}
int CV9_BATCH_UPDlg::count_md5(char *md5)
{
char file[200]= {0};
sprintf(file, "%s.tar.gz", UPDATEDIR""UPDATENAME);
memset(md5, 0, sizeof(md5));
file_md5(file, md5);
g_file_len = file_len(file);
//char buf[100] = {0};
//sprintf(buf, "tcp_num:%d", len);
//AfxMessageBox(buf);
return 0;
}
int CV9_BATCH_UPDlg::count_update_num()
{
DEVINFOLIST * p = m_pHostUpdate->pNext;
CString str;
int index = 0;
while(p != NULL)
{
p = p->pNext;
index++;
}
return index;
}
DEVINFOLIST * CV9_BATCH_UPDlg::first_update(DEVINFOLIST *p ,int num)
{
while(p != NULL)
{
if(num == 0)
{
break;
}
p = p->pNext;
num--;
}
return p;
}
DEVINFOLIST * CV9_BATCH_UPDlg::next_update(DEVINFOLIST *p ,int num)
{
int totle = update_num;
while(p != NULL)
{
if(totle == 0)
{
break;
}
p = p->pNext;
totle--;
}
return p;
}
int CV9_BATCH_UPDlg::update_rec_flag(int num, const char *ip, int index)
{
char buf[100] = {0};
switch (num)
{
case UP_START:
sprintf(buf, "板卡:%s 发送url成功", ip);
status[index] = UP_START;
CProgressCtrlUpdate(1, buf);
case UP_TRANS_SUC:
sprintf(buf, "板卡:%s 文件下载成功", ip);
status[index] = UP_TRANS_SUC;
CProgressCtrlUpdate(1, buf);
return 1;
case UP_TRANS_ERR:
sprintf(buf, "板卡:%s 文件下载失败", ip);
CProgressCtrlUpdate(1, buf);
return 0;
case UP_MD5_SUC:
sprintf(buf, "板卡:%s MD5校验成功", ip);
status[index] = UP_MD5_SUC;
//AfxMessageBox(buf);
CProgressCtrlUpdate(1, buf);
return 1;
case UP_MD5_ERR:
sprintf(buf, "板卡:%s MD5校验失败", ip);
//AfxMessageBox(buf);
CProgressCtrlUpdate(1, buf);
return 0;
case UP_UPDATE_SUC:
sprintf(buf, "板卡:%s 更新文件成功", ip);
status[index] = UP_UPDATE_SUC;
//AfxMessageBox(buf);
CProgressCtrlUpdate(1, buf);
return 0;
case UP_UPDATE_ERR:
sprintf(buf, "板卡:%s 更新文件失败", ip);
//AfxMessageBox(buf);
CProgressCtrlUpdate(1, buf);
return 0;
}
return -1;
}
int CV9_BATCH_UPDlg::tcp_send(int num)
{
DEVINFOLIST * p = m_pHostUpdate->pNext;
int index = 0;
int index_totle = num;
char buf[100] = {0};
CString str;
SOCKET sockSrv;
SOCKADDR_IN servaddr;
unsigned int len;
unsigned long long send_len;
char filename[100] = {0};
sprintf(filename, "%s.tar.gz", UPDATEDIR""UPDATENAME);
FILE *fp;
char buf_send[BUFFSIZE] = {0};
int read_len;
int ret;
char statusbuf[10] = {0};
int status_update;
int timeout = 1000*60*2;
int sleep_flag = 0;
p = first_update(p ,num);
while(p != NULL)
{
send_len = 0;
sprintf(buf, "正在升级板卡:%s", p->ip);
CProgressCtrlUpdate(1, buf);
//AfxMessageBox(buf);
sockSrv = socket(AF_INET, SOCK_STREAM, 0);
if (sockSrv == INVALID_SOCKET)
{
str.Format ("%s", "socket error");
AfxMessageBox(str);
return -1;
}
if(setsockopt(sockSrv,SOL_SOCKET,SO_RCVTIMEO,(char *)&timeout,sizeof(timeout)))
{
AfxMessageBox("设置超时时间出错");
}
memset(&servaddr, 0, sizeof(servaddr));
servaddr.sin_family = AF_INET;
servaddr.sin_port = htons(BATCH_UP_PORT_C);
servaddr.sin_addr.s_addr = inet_addr(p->ip);
if(connect(sockSrv, (SOCKADDR *)&servaddr, sizeof(servaddr)) == SOCKET_ERROR)
{
str.Format ("%s", "connect error");
AfxMessageBox(str);
//fclose(fp);
closesocket(sockSrv);
return -1;
}
//update_type(1type)+md5(32byte)+filelen(8byte)+files
if(send_pack(sockSrv, "1", 1, 0) < 0)
{
AfxMessageBox("send md5 error");
//fclose(fp);
closesocket(sockSrv);
return -1;
}
if(send_pack(sockSrv, md5, 32, 0) < 0)
{
AfxMessageBox("send md5 error");
//fclose(fp);
closesocket(sockSrv);
return -1;
}
//AfxMessageBox(md5);
//str.Format ("%llu", g_file_len &0x00000000ffffffff);
//AfxMessageBox(str);
len = (g_file_len >> 32) & 0x00000000ffffffff;
len = htonl(len);
//str.Format ("%u", len);
//AfxMessageBox(str);
if(send_pack(sockSrv, (char *)&len, 4, 0) < 0)
{
AfxMessageBox("send file len error");
//fclose(fp);
closesocket(sockSrv);
return -2;
}
len = (g_file_len & 0x00000000ffffffff);
len = htonl(len);
//str.Format ("%u", len);
//AfxMessageBox(str);
if(send_pack(sockSrv, (char *)&len, 4, 0) < 0)
{
AfxMessageBox("send file len error");
//fclose(fp);
closesocket(sockSrv);
return -2;
}
fp = fopen(filename, "rb");
if(fp == NULL)
{
AfxMessageBox("fopen file error");
//fclose(fp);
closesocket(sockSrv);
return -3;
}
memset(buf_send, 0, BUFFSIZE);
sleep_flag = 0;
while((read_len = fread(buf_send, sizeof(char), BUFFSIZE, fp)) > 0)
{
//str.Format ("%u", read_len);
//AfxMessageBox(str);
if((ret = send_pack(sockSrv, buf_send, read_len, 0)) < 0)
{
AfxMessageBox("send file error");
fclose(fp);
closesocket(sockSrv);
return -4;
}
if(sleep_flag > 1024*100)
{
sleep_flag = 0;
Sleep(5000);
}
sleep_flag++;
send_len += read_len;
memset(buf_send, 0, BUFFSIZE);
}
if(send_len != g_file_len)
{
AfxMessageBox("发送文件大小与文件实际不符");
fclose(fp);
closesocket(sockSrv);
return -5;
}
sprintf(buf, "板卡:%s 发送升级文件成功,请等待板卡升级...", p->ip);
CProgressCtrlUpdate(1, buf);
fclose(fp);
shutdown(sockSrv, SD_SEND);
memset(statusbuf, 0, sizeof(statusbuf));
//Sleep(10000);
//return "status:ret"
if((ret = recv(sockSrv, statusbuf, sizeof(statusbuf), 0)) > 0)
{
if(strncmp(statusbuf, "status:", 7) != 0)
{
//closesocket(sockSrv);
AfxMessageBox("板卡返回数据有误");
index++;
index_totle += update_num;
p = next_update(p, num);
closesocket(sockSrv);
continue;
}
if(strlen(statusbuf) < 9)
{
//closesocket(sockSrv);
AfxMessageBox("板卡返回数据有误");
index++;
index_totle += update_num;
p = next_update(p, num);
closesocket(sockSrv);
continue;
}
status_update = atoi(statusbuf+7);
update_rec_flag(status_update, p->ip, index_totle);
}
if (ret < 0)
{
ret = WSAGetLastError();
closesocket(sockSrv);
if(ret == WSAETIMEDOUT)
{
sprintf(buf, "板卡:%s 升级状态超时...", p->ip);
AfxMessageBox(buf);
}
else
{
sprintf(buf, "板卡:%s 接收状态出错...", p->ip);
AfxMessageBox(buf);
}
index++;
index_totle += update_num;
p = next_update(p, num);
continue;
}
closesocket(sockSrv);
index++;
index_totle += update_num;
p = next_update(p, num);
}
return 0;
}
int CV9_BATCH_UPDlg::tcp_update()
{
int tcp_num = 1;
int tcp_flag = 1;
int flag = 1; //1-suc 0-err
int pro_num;
int board_num = 0;
memset(status, 0, sizeof(status));
CEdit* pBoxOne;
CString str;
char *p;
pBoxOne = (CEdit*)GetDlgItem(IDC_EDIT2);
pBoxOne-> GetWindowText(str);
p = str.GetBuffer();
if (strlen(p) != 0)
{
tcp_num = atoi(p);
}
else
{
tcp_flag = 0;
}
if(tcp_num<1 || tcp_num > THREAD_MAX)
{
AfxMessageBox("tcp并发数只能在1~8之间");
return 0;
}
update_num = count_update_num();
board_num = update_num;
//char buf[100] = {0};
//sprintf(buf, "update_num:%d", update_num);
//AfxMessageBox(buf);
if (update_num == 0)
{
AfxMessageBox("请选择需要升级的板卡");
return 0;
}
//sprintf(buf, "tcp_num:%d", tcp_num);
//AfxMessageBox(buf);
if ((tcp_flag != 0) && (update_num > tcp_num))
{
update_num = tcp_num;
}
memset(m_file_cvs, 0, sizeof(m_file_cvs));
CreateCSV(m_file_cvs);
//char buf[100] = {0};
//sprintf(buf, "tcp_num:%d", tcp_num);
//AfxMessageBox(buf);
pro_num = 3+update_num*4;
CProgressCtrlInit(pro_num);
tar_gz();
count_md5(md5);
CProgressCtrlUpdate(1, "整备发送升级包...");
//AfxMessageBox(md5);
HANDLE hThread[8];
g_hThreadParameter = CreateSemaphore(NULL, 0, 1, NULL);//当前0个资源,最大允许1个同时访问
int i;
for(i=0;i<board_num;i++)
{
g_status[i] = CreateSemaphore(NULL, 0, 1, NULL);
}
for(i=0;i<update_num;i++)
{
hThread[i] = (HANDLE)_beginthreadex(NULL, 0, tcp_client, &i, 0, NULL);
WaitForSingleObject(g_hThreadParameter, INFINITE);//等待信号量>0
}
int nIndex = WaitForMultipleObjects(update_num, hThread, TRUE,INFINITE);
if(nIndex == WAIT_FAILED)
{
AfxMessageBox("线程退出出错");
}
else if((nIndex >= WAIT_OBJECT_0) && (nIndex < tcp_num))
{
//AfxMessageBox("线程退出正常");
}
CloseHandle(g_hThreadParameter);
for(i=0;i<update_num;i++)
{
CloseHandle( hThread[i] );
}
int j =0 ;
for(i=0;i<board_num;i++)
{
if( status[i] == UP_MD5_SUC)
{
g_status_check[j] = g_status[i];
j++;
}
}
char buf[100];
if(j > 0)
{
nIndex = WaitForMultipleObjects(j, g_status_check, TRUE, 60*60*1000);
if(nIndex == WAIT_FAILED)
{
sprintf(buf, "等待更新状态出错 %d", j);
AfxMessageBox(buf);
}
else if((nIndex >= WAIT_OBJECT_0) && (nIndex < j))
{
//AfxMessageBox("线程退出正常");
}
}
for(i=0;i<board_num;i++)
{
CloseHandle(g_status[i]);
}
for(i=0;i<board_num;i++)
{
if( status[i] != UP_UPDATE_SUC)
{
flag = 0;
break;
}
}
AddToCSV(m_file_cvs, m_pHostUpdate->pNext, status);
int num;
CProgressCtrl *pProgCtrl=(CProgressCtrl*)GetDlgItem(IDC_PROGRESS1);
num = pro_num - pProgCtrl->GetPos();
if(flag == 1)
CProgressCtrlUpdate(num, "升级成功");
else
CProgressCtrlUpdate(num, "升级失败");
return 0;
}
int CV9_BATCH_UPDlg::ftp_update()
{
DEVINFOLIST * p = m_pHostUpdate->pNext;
SOCKET sockSrv;
SOCKADDR_IN servaddr;
CString str;
char ftp_url[100] = {0};
CEdit* pBoxOne;
pBoxOne = (CEdit*)GetDlgItem(IDC_EDIT1);
pBoxOne-> GetWindowText(str);
int timeout = 30000;
int flag = 1;
char statusbuf[10] = {0};
int status_update;
int ret;
int index = 0;
char buf[100] = {0};
int board_num;
//GetDlgItem(IDC_STATIC11)->SetWindowText("正在发送URL到各板卡..");
memset(status, 0, sizeof(status));
update_num = count_update_num();
board_num = update_num;
if (update_num == 0)
{
AfxMessageBox("请选择需要升级的板卡");
return 0;
}
if(strlen(str.GetBuffer()) == 0)
{
AfxMessageBox("请输入正确的url");
return 0;
}
strcpy(ftp_url, str.GetBuffer());
char *pstr = strstr(ftp_url, "//");
if(pstr == NULL)
{
AfxMessageBox("请输入正确的url");
return 0;
}
pstr = strchr(ftp_url, ':');
if(pstr == NULL)
{
AfxMessageBox("请输入正确的url");
return 0;
}
pstr = strchr(ftp_url, '@');
if(pstr == NULL)
{
AfxMessageBox("请输入正确的url");
return 0;
}
CreateCSV(m_file_cvs);
int pro_num = 3*update_num + 2;
CProgressCtrlInit(pro_num);
CProgressCtrlUpdate(1, "整备发送ftp url...");
int i;
for(i=0;i<board_num;i++)
{
g_status[i] = CreateSemaphore(NULL, 0, 1, NULL);
}
while(p != NULL)
{
//AfxMessageBox(buf);
sockSrv = socket(AF_INET, SOCK_STREAM, 0);
if (sockSrv == INVALID_SOCKET)
{
str.Format ("%s", "socket error");
AfxMessageBox(str);
return -1;
}
setsockopt(sockSrv,SOL_SOCKET,SO_RCVTIMEO,(char *)&timeout,sizeof(timeout));
memset(&servaddr, 0, sizeof(servaddr));
servaddr.sin_family = AF_INET;
servaddr.sin_port = htons(BATCH_UP_PORT_C);
servaddr.sin_addr.s_addr = inet_addr(p->ip);
if(connect(sockSrv, (SOCKADDR *)&servaddr, sizeof(servaddr)) == SOCKET_ERROR)
{
str.Format ("%s", "connect error");
AfxMessageBox(str);
//fclose(fp);
closesocket(sockSrv);
return -1;
}
//update_type(1byte)+ftpurl(100byte)
//1-tcp 2-ftp
if(send_pack(sockSrv, "2", 1, 0) < 0)
{
AfxMessageBox("send md5 error");
//fclose(fp);
closesocket(sockSrv);
return -1;
}
if(send_pack(sockSrv, ftp_url, sizeof(ftp_url), 0) < 0)
{
AfxMessageBox("send ftp_url error");
//fclose(fp);
closesocket(sockSrv);
return -1;
}
//sprintf(buf, "板卡:%s 发送ftp url 成功", p->ip);
update_rec_flag(UP_START, p->ip, index);
#if 0
memset(statusbuf, 0, sizeof(statusbuf));
while((ret = recv(sockSrv, statusbuf, sizeof(statusbuf), 0)) > 0)
{
if(strncmp(statusbuf, "status:", 7) != 0)
{
closesocket(sockSrv);
break;
}
if(strlen(statusbuf) < 9)
{
closesocket(sockSrv);
break;
}
status_update = atoi(statusbuf+7);
if(update_rec_flag(status_update, p->ip, index) == 0)
break;
}
if(ret < 0)
{
closesocket(sockSrv);
AfxMessageBox("接收板卡升级状态超时或者错误");
}
#endif
closesocket(sockSrv);
p = p->pNext;
index++;
}
int j =0 ;
for(i=0;i<index;i++)
{
if( (status[i] == UP_START) || (status[i] == UP_TRANS_SUC ))
{
g_status_check[j] = g_status[i];
j++;
}
}
//char buf[100];
if(j > 0)
{
ret = WaitForMultipleObjects(j, g_status_check, TRUE, 60*60*1000);
if(ret == WAIT_FAILED)
{
sprintf(buf, "等待更新状态出错 %d", j);
AfxMessageBox(buf);
}
else if((ret >= WAIT_OBJECT_0) && (ret < j))
{
//AfxMessageBox("线程退出正常");
}
}
for(i=0;i<board_num;i++)
{
CloseHandle(g_status[i]);
}
for(i=0;i<index;i++)
{
if( status[i] != UP_UPDATE_SUC)
{
flag = 0;
break;
}
}
AddToCSV(m_file_cvs, m_pHostUpdate->pNext, status);
int num;
CProgressCtrl *pProgCtrl=(CProgressCtrl*)GetDlgItem(IDC_PROGRESS1);
num = pro_num - pProgCtrl->GetPos();
if(flag == 1)
CProgressCtrlUpdate(num, "升级成功");
else
CProgressCtrlUpdate(num, "升级失败");
//GetDlgItem(IDC_STATIC11)->SetWindowText("发送URL到各板卡完成...");
return 0;
}
void CV9_BATCH_UPDlg::OnBnClickedOk()
{
// TODO: 在此添加控件通知处理程序代码
((CButton*)GetDlgItem(IDOK))->EnableWindow(FALSE);
((CButton*)GetDlgItem(IDC_BUTTON1))->EnableWindow(FALSE);
((CButton*)GetDlgItem(IDC_BUTTON2))->EnableWindow(FALSE);
HANDLE hThread = (HANDLE)_beginthreadex(NULL, 0, updatemain, NULL, 0, NULL);
CloseHandle( hThread );
//OnOK();
}
<file_sep>#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <unistd.h>
#include <netdb.h>
#include <sys/mman.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <errno.h>
#include <pthread.h>
#include <sys/ioctl.h>
#include <net/if.h>
#include <netinet/in.h>
#include <linux/sockios.h>
#define BATCH_UP_PORT 20000
#define BATCH_UP_PORT_C 20001
#define BATCH_STATUS_PORT 20002
#define BATCH_UP_IP "192.168.110.119"
#define ROUTE_PORT 60001
#define ROUTE_IP "192.168.111.1"
#define BUFFSIZE 1024
#define FILE_DIR "/mnt/cdrom/updates/"
#define MD5_FILE "md5.file"
#define FILE_NAME "updates.tar.gz"
#define TMP_FILE "/tmp/tmp.file"
#define BS_VERSION_FILE "/mnt/cdrom/wibox/web/WEB-INF/classes/wibox_config.properties"
#define BS_DIR "/mnt/cdrom/wibox"
int write_appV();
int write_recordV();
int restart_flag = 0;
enum update_status
{
UP_START,
UP_TRANS_SUC = 10,
UP_TRANS_ERR,
UP_MD5_SUC = 20,
UP_MD5_ERR,
UP_UPDATE_SUC = 30,
UP_UPDATE_ERR
};
struct DEVINFO
{
char name[48];
int type;
char routeV[16];
char kernelV[16];
char appV[16];
char recordV[16];
char bsV[16];
char str1[16];
char str2[16];
};
struct DEVINFO devinfo;
void* send_devinfo(void *arg)
{
unsigned char buf[1024] = {0};
int sockfd;
struct sockaddr_in servaddr;
bzero(&servaddr, sizeof(servaddr));
servaddr.sin_family = AF_INET;
servaddr.sin_port = htons(BATCH_UP_PORT);
inet_aton(BATCH_UP_IP, &servaddr.sin_addr);
printf("send_devinfo start\n");
if((sockfd = socket(AF_INET,SOCK_DGRAM,0)) < 0)
{
perror("send_devinfo, socket:");
return NULL;
}
if(connect(sockfd, (struct sockaddr *)&servaddr, sizeof(servaddr)) < 0)
{
perror("send_devinfo, connect:");
return NULL;
}
while(1)
{
//printf("start connect\n");
memcpy(buf, devinfo.name, sizeof(devinfo.name));
int tmp = htonl(devinfo.type);
int index = sizeof(devinfo.name);
memcpy(buf+index, &tmp, sizeof(int));
index += sizeof(int);
memcpy(buf+index, devinfo.routeV, sizeof(devinfo.routeV));
index += sizeof(devinfo.routeV);
memcpy(buf+index, devinfo.kernelV, sizeof(devinfo.kernelV));
index += sizeof(devinfo.kernelV);
memcpy(buf+index, devinfo.appV, sizeof(devinfo.appV));
index += sizeof(devinfo.appV);
memcpy(buf+index, devinfo.recordV, sizeof(devinfo.recordV));
index += sizeof(devinfo.recordV);
memcpy(buf+index, devinfo.bsV, sizeof(devinfo.bsV));
index += sizeof(devinfo.bsV);
memcpy(buf+index, devinfo.str1, sizeof(devinfo.str1));
index += sizeof(devinfo.str1);
memcpy(buf+index, devinfo.str2, sizeof(devinfo.str2));
//sendto(sockfd, buf, sizeof(devinfo), 0, (struct sockaddr *)&servaddr, sizeof(servaddr));
write(sockfd, buf, sizeof(devinfo));
sleep(1);
}
close(sockfd);
printf("send_devinfo end\n");
return NULL;
}
int md5_check(const char *file, const char *md5)
{
char cmd[100] = {0};
char md5_count[50] = {0};
char md5_file_name[100] = {0};
sprintf(md5_file_name, "%s%s", FILE_DIR, MD5_FILE);
sprintf(cmd, "md5sum %s > %s", file, md5_file_name);
system(cmd);
FILE *fp = fopen(md5_file_name, "r");
fscanf(fp, "%s", md5_count);
fclose(fp);
printf("md5 trans:%s\n", md5);
printf("md5 count:%s\n", md5_count);
return strncmp(md5_count, md5, 32);
}
int find_name(char *file, char *filein)
{
char cmd[100] = {0};
sprintf(cmd, "ls -lt %s|grep %s|head -n 1|awk '{print $NF}' > %s", FILE_DIR, filein, TMP_FILE);
system(cmd);
FILE *fp = fopen(TMP_FILE, "r");
if(fp == NULL)
{
return -1;
}
fgets(file, 80, fp);
char *p = file;
while(*p != '\0')
{
if(*p == '\n')
*p = '\0';
p++;
}
fclose(fp);
return 0;
}
int find_name_tar(char *file, char *filein)
{
char cmd[100] = {0};
sprintf(cmd, "tar tvf %s%s | awk '{print $NF}' > %s", FILE_DIR, filein, TMP_FILE);
//printf(cmd);
system(cmd);
FILE *fp = fopen(TMP_FILE, "r");
if(fp == NULL)
{
return -1;
}
fgets(file, 80, fp);
char *p = file;
while(*p != '\0')
{
if(*p == '\n')
*p = '\0';
p++;
}
fclose(fp);
return 0;
}
int find_name_app(char *file, char *filein, char *app)
{
char cmd[100] = {0};
sprintf(cmd, "tar tvf %s%s | grep %s | awk '{print $NF}' > %s", FILE_DIR, file, app, TMP_FILE);
system(cmd);
FILE *fp = fopen(TMP_FILE, "r");
if(fp == NULL)
{
return -1;
}
fgets(file, 80, fp);
char *p = file;
while(*p != '\0')
{
if(*p == '\n')
*p = '\0';
p++;
}
fclose(fp);
return 0;
}
int update_route()
{
char file[100] = {0};
char file_x[200] = {0};
if(find_name(file, "route") == 0)
{
if(strlen(file) == 0)
{
printf("updates.tar.gz has no route.tar.\n");
return 0;
}
}
else
{
return -2;
}
if(find_name_tar(file_x, file) == 0)
{
if(strlen(file_x) == 0)
{
printf("route.tar has no file.\n");
return -1;
}
char cmd[300] = {0};
sprintf(cmd, "tar xvf %s%s -C %s", FILE_DIR, file, FILE_DIR);
//printf(cmd);
system(cmd);
memset(cmd, 0, sizeof(cmd));
sprintf(cmd, "/root/package_push --file %s%s --ip %s", FILE_DIR, file_x, ROUTE_IP);
int ret = system(cmd);
if(ret == -1)
{
printf("system error\n");
return -2;
}
if(WIFEXITED(ret))
{
if(WEXITSTATUS(ret) == 0)
{
//printf("cmd success\n");
}
else
{
printf("cmd error\n");
return -3;
}
}
else
{
printf("shell error\n");
return -4;
}
}
return 0;
}
int update_kernel()
{
char file[100] = {0};
char file_x[100] = {0};
if(find_name(file, "kernel") == 0)
{
if(strlen(file) == 0)
{
printf("updates.tar.gz has no kernel.tar.\n");
return 0;
}
}
else
{
return -2;
}
char cmd[100] = {0};
sprintf(cmd, "tar xvf %s%s -C %s", FILE_DIR, file, FILE_DIR);
//printf(cmd);
system(cmd);
if(find_name_tar(file_x, file) == 0)
{
if(strlen(file_x) == 0)
{
printf("kernel.tar has no file.\n");
return -1;
}
memset(cmd, 0, sizeof(cmd));
sprintf(cmd, "dd if=%s%s of=/dev/mmcblk0p5 bs=1024k count=8", FILE_DIR, file_x);
system(cmd);
}
else
return -3;
return 0;
}
int update_app()
{
char file[100] = {0};
char version[20] = {0};
if(find_name(file, "app") == 0)
{
if(strlen(file) == 0)
{
printf("updates.tar.gz has no app.tar.\n");
return 0;
}
}
else
{
return -2;
}
char cmd[200] = {0};
//printf("file :%s\n", file);
printf("updating V9-Wibox or V9-StreamMonitor will kill their process\n");
sprintf(cmd, "killall start_wdg;killall V9-Wibox;killall V9-StreamMonitor;killall V9-IpMonitor");
system(cmd);
memset(cmd, 0, sizeof(cmd));
sprintf(cmd, "tar xvf %s%s -C %s", FILE_DIR, file, "/root/");
system(cmd);
memset(cmd, 0, sizeof(cmd));
sprintf(cmd, "cd /root/;mv *.so /usr/lib/; chmod +x *");
system(cmd);
//printf("file :%s\n", file);
char *p = strstr(file, ".tar");
if(p == NULL)
{
printf("file name error\n");
return -3;
}
memcpy(version, file+4, p-file-4);
write_appV(version, devinfo.type);
#if 0
if(find_name_app(file, "app", "V9-Wibox") == 0)
{
if(strlen(file) != 0)
{
printf("V9-Wibox update\n");
write_appV(version, 0x21);
}
}
if(find_name_app(file, "app", "V9-StreamMonitor") == 0)
{
if(strlen(file) != 0)
{
printf("V9-StreamMonitor update\n");
write_appV(version, 0x12);
}
}
#endif
return 0;
}
int update_record()
{
char file[100] = {0};
char version[20] = {0};
if(find_name(file, "record") == 0)
{
if(strlen(file) == 0)
{
printf("updates.tar.gz has no record.tar.\n");
return 0;
}
}
else
{
return -2;
}
char cmd[100] = {0};
sprintf(cmd, "tar xvf %s%s -C %s", FILE_DIR, file, FILE_DIR);
system(cmd);
memset(cmd, 0, sizeof(cmd));
sprintf(cmd, "killall Record");
system(cmd);
memset(cmd, 0, sizeof(cmd));
sprintf(cmd, "cd %s;cp Record /mnt/cdrom/record/Record;chmod +x /mnt/cdrom/record/Record", FILE_DIR);
system(cmd);
char *p = strstr(file, ".tar");
if(p == NULL)
return -3;
memcpy(version, file+7, p-file-7);
write_recordV(version);
return 0;
}
int update_bs()
{
char file[100] = {0};
if(find_name(file, "bs") == 0)
{
if(strlen(file) == 0)
{
printf("updates.tar.gz has no bs.tar.\n");
return 0;
}
}
else
{
return -2;
}
char cmd[100] = {0};
sprintf(cmd, "mkdir -p %s;killall nginx;killall java;tar xvf %s%s -C %s", BS_DIR,FILE_DIR, file, BS_DIR);
system(cmd);
return 0;
}
int tar_gzip_x(const char *file)
{
char cmd[100] = {0};
sprintf(cmd, "tar zxvf %s -C %s", file, FILE_DIR);
int ret = system(cmd);
if(ret == -1)
{
printf("system error\n");
return -2;
}
if(WIFEXITED(ret))
{
if(WEXITSTATUS(ret) == 0)
{
//printf("cmd success\n");
}
else
{
printf("cmd error\n");
return -3;
}
}
else
{
printf("shell error\n");
return -4;
}
return 0;
}
int clear_file()
{
char cmd[100] = {0};
printf("clear dir:%s\n", FILE_DIR);
sprintf(cmd, "rm -f %s*", FILE_DIR);
system(cmd);
return 0;
}
int updatesfile(const char *file)
{
int ret;
ret = tar_gzip_x(file);
if(ret != 0)
{
printf("tar zxvf error\n");
return -1;
}
if(update_route())
{
printf("update_route error\n");
return -2;
}
restart_flag = 1;
if(update_kernel())
{
printf("update_kernel error\n");
return -3;
}
if(update_app())
{
printf("update_app error\n");
return -4;
}
if(update_record())
{
printf("update_record error\n");
return -5;
}
if(update_bs())
{
printf("update_bs error\n");
return -6;
}
printf("updates success\n");
return 0;
}
int ftp_rec(const char *ftp_url)
{
char user[20] = {0};
char passwd[20] = {0};
char port[6] = {0};
char ip[16] = {0};
char *ps = strstr(ftp_url, "//");
if(ps == NULL)
{
printf("ftp url error\n");
return -1;
}
char *pe = strchr(ps, ':');
if(pe == NULL)
{
printf("ftp url error\n");
return -1;
}
memcpy(user, ps+2, pe-ps-2);
printf("user:%s\n", user);
ps = pe;
pe = strchr(ftp_url, '@');
if(pe == NULL)
{
printf("ftp url error\n");
return -1;
}
memcpy(passwd, ps+1, pe-ps-1);
printf("passwd:%s\n", passwd);
ps = pe;
pe = strchr(ps, ':');
if(pe == NULL)
{
printf("ftp url has no port, use default port 21\n");
strcpy(port, "21");
strcpy(ip, ps+1);
printf("ip:%s\n", ip);
}
else
{
memcpy(ip, ps+1, pe-ps-1);
printf("ip:%s\n", ip);
ps = pe;
strcpy(port, ps+1);
printf("port:%s\n", port);
}
char cmd[100] = {0};
sprintf(cmd, "cd %s;ftpget -u %s -p %s -P %s %s %s", FILE_DIR, user, passwd, port, ip, FILE_NAME);
int ret = system(cmd);
if(ret == -1)
{
printf("system error\n");
return -2;
}
if(WIFEXITED(ret))
{
if(WEXITSTATUS(ret) == 0)
{
printf("cmd success\n");
}
else
{
printf("cmd error\n");
return -3;
}
}
else
{
printf("shell error\n");
return -4;
}
return 0;
}
int ftp_update()
{
int ret;
char file[100] = {0};
sprintf(file, "%s", FILE_DIR""FILE_NAME);
ret = tar_gzip_x(file);
if(ret != 0)
{
printf("tar zxvf error\n");
return -1;
}
if(update_route())
{
printf("update_route error\n");
return -2;
}
restart_flag = 1;
if(update_kernel())
{
printf("update_kernel error\n");
return -3;
}
if(update_app())
{
printf("update_app error\n");
return -4;
}
if(update_record())
{
printf("update_record error\n");
return -5;
}
if(update_bs())
{
printf("update_bs error\n");
return -6;
}
return 0;
}
int send_update_flag(char *flag)
{
int sockfd;
struct sockaddr_in servaddr;
bzero(&servaddr, sizeof(servaddr));
servaddr.sin_family = AF_INET;
servaddr.sin_port = htons(BATCH_STATUS_PORT);
inet_aton(BATCH_UP_IP, &servaddr.sin_addr);
int times =0;
while(1)
{
times ++;
if((sockfd = socket(AF_INET,SOCK_STREAM,0)) < 0)
{
perror("send_update flag, socket");
return -1;
}
if(connect(sockfd, (struct sockaddr *)&servaddr, sizeof(servaddr)) < 0)
{
perror("send_update flag, connect");
close(sockfd);
if(times > 5)
{
return -2;
}
sleep(5);
continue;
}
if(send(sockfd, flag, 10, 0) < 0)
{
perror("send_update flag, send");
close(sockfd);
return -1;
}
close(sockfd);
return 0;
}
return 0;
}
void* update_thread(void *arg)
{
int ser_sock, cli_sock;
socklen_t cli_len;
unsigned int len;
int recv_len;
unsigned long long file_len, recv_file_len;
struct sockaddr_in ser_addr, cli_addr;
char md5[50] = {0};
char filename[100] = {0};
char status_update[10] = {0};
char buf[BUFFSIZE] = {0};
char update_type[2] = {0};
char ftp_url[100] = {0};
sprintf(filename, "%s", FILE_DIR""FILE_NAME);
FILE *fp;
int ret;
struct timeval tv;
tv.tv_sec = 30;
tv.tv_usec = 0;
printf("update_thread start\n");
if((ser_sock = socket(AF_INET,SOCK_STREAM,0)) < 0)
{
perror("socket error:");
return NULL;
}
memset(&ser_addr, 0, sizeof(ser_addr));
ser_addr.sin_family = AF_INET;
ser_addr.sin_port = htons(BATCH_UP_PORT_C);
ser_addr.sin_addr.s_addr = htons(INADDR_ANY);
printf("bind ...\n");
if(bind(ser_sock, (struct sockaddr *)&ser_addr, sizeof(ser_addr)) == -1)
{
perror("bind, error:");
return NULL;
}
printf("listen ...\n");
if(listen(ser_sock, 10) == -1)
{
perror("listen, error:");
return NULL;
}
while(1)
{
cli_len = sizeof(cli_addr);
printf("accept ...\n");
cli_sock = accept(ser_sock, (struct sockaddr *)&cli_addr, &cli_len);
if(cli_sock == -1)
{
perror("accept, error:");
//close(cli_sock);
//fclose(fp);
continue;
}
ret=setsockopt(cli_sock,SOL_SOCKET,SO_RCVTIMEO,&tv,sizeof(tv));
printf("client %s connect to the server\n", inet_ntoa(cli_addr.sin_addr));
if(recv(cli_sock, update_type, 1, 0) != 1)
{
perror("recv update_type error");
close(cli_sock);
//fclose(fp);
continue;
}
//1-tcp 2-ftp
printf("update_type:%s\n", update_type);
//ftp
if(atoi(update_type) == 2)
{
if(recv(cli_sock, ftp_url, 100, 0) != 100)
{
perror("recv ftp url error");
close(cli_sock);
//fclose(fp);
continue;
}
//close(cli_sock);
close(cli_sock);
clear_file();
printf("ftp url:%s\n", ftp_url);
ret = ftp_rec(ftp_url);
if(ret == 0)
{
printf("ftp download file suc\n");
//sprintf(status_update, "status:%d", UP_TRANS_SUC);
sprintf(status_update, "status:%d", UP_TRANS_SUC);
}else
{
printf("ftp download file error\n");
//sprintf(status_update, "status:%d", UP_TRANS_ERR);
sprintf(status_update, "status:%d", UP_TRANS_ERR);
}
if(send_update_flag(status_update))
{
printf("send status(ftp download file) error\n");
}
restart_flag = 0;
ret = ftp_update();
clear_file();
if(ret == 0)
{
printf("ftp update suc\n");
sprintf(status_update, "status:%d", UP_UPDATE_SUC);
}else
{
printf("ftp update error\n");
sprintf(status_update, "status:%d", UP_UPDATE_ERR);
}
if(send_update_flag(status_update))
{
printf("send status(ftp updates file) error\n");
}
#if 0
if(send(cli_sock, status_update, sizeof(status_update), 0) < 0)
{
perror("send status error");
close(cli_sock);
continue;
}
#endif
if(restart_flag == 1)
{
printf("the system will restart...\n");
system("reboot");
}
continue;
}
//tcp
if(recv(cli_sock, md5, 32, 0) != 32)
{
perror("recv md5 error:");
close(cli_sock);
//fclose(fp);
continue;
}
printf("md5:%s\n", md5);
if(recv(cli_sock, (char *)&len, 4, 0) != 4)
{
perror("recv file len error:");
close(cli_sock);
//fclose(fp);
continue;
}
len = ntohl(len);
//printf("%u\n", len);
file_len = ((unsigned long long)len << 32);
if(recv(cli_sock, (char *)&len, 4, 0) != 4)
{
perror("recv file len error:");
close(cli_sock);
//fclose(fp);
continue;
}
len = ntohl(len);
//printf("%u\n", len);
file_len += len;
printf("file_len:%llu\n", file_len);
printf("filename :%s\n", filename);
clear_file();
fp = fopen(filename, "w");
memset(buf, 0, BUFFSIZE);
recv_file_len = 0;
while((recv_len = recv(cli_sock, buf, BUFFSIZE, 0)) > 0)
{
recv_file_len += recv_len;
//printf("%d %llu %x %x\n", recv_len, recv_file_len, buf[0], buf[1]);
if(fwrite(buf, sizeof(char), recv_len, fp) < recv_len)
{
perror("recv file len error");
break;
}
memset(buf, 0, BUFFSIZE);
}
if(recv_len < 0)
{
if(errno == EWOULDBLOCK)
{
perror("recv file time out");
close(cli_sock);
fclose(fp);
continue;
}
}
if(recv_file_len != file_len)
{
printf("recv file len is diffent from file len\n");
close(cli_sock);
fclose(fp);
continue;
}
fclose(fp);
if(md5_check(filename, md5) != 0)
{
printf("md5 checking is not right");
sprintf(status_update, "status:%d", UP_MD5_ERR);
send(cli_sock, status_update, sizeof(status_update), 0);
close(cli_sock);
continue;
}
printf("send status md5\n");
//sleep(30);
//printf("sleep end\n");
sprintf(status_update, "status:%d", UP_MD5_SUC);
if(send(cli_sock, status_update, sizeof(status_update), 0) < 0)
{
perror("send status error");
close(cli_sock);
continue;
}
close(cli_sock);
restart_flag = 0;
if(updatesfile(filename) == 0)
{
//clear_file();
printf("updates status success\n");
sprintf(status_update, "status:%d", UP_UPDATE_SUC);
}
else
{
//clear_file();
printf("updates status error\n");
sprintf(status_update, "status:%d", UP_UPDATE_ERR);
}
if(send_update_flag(status_update))
{
printf("send updates flag error\n");
}
if(restart_flag == 1)
{
printf("the system will restart...\n");
system("reboot");
}
}
return NULL;
}
void mk_updates_dir()
{
char cmd[100] = {0};
sprintf(cmd, "mkdir -p %s", FILE_DIR);
system(cmd);
}
int read_name(char *kernelV)
{
FILE *fp = NULL;
char *p;
char cmd[100] = {0};
char val[100] = {0};
sprintf(cmd, "uname -a | awk '{print $3}' > /tmp/wibox.name");
system(cmd);
fp = fopen ( "/tmp/wibox.name", "rb" );
if(fp == NULL)
return -1;
fgets(val, 80, fp);
p = val;
while(*p != '\0')
{
if(*p == '\n')
*p = '\0';
p++;
}
fclose(fp);
system("rm /tmp/wibox.name");
//printf("%s\n", val);
p = strstr(val, "SNM");
if(p)
{
memcpy(kernelV, val, p-1-val);
return (p[4]-'0');
}
else
return 1;
}
int read_type()
{
FILE *fp = NULL;
char *p;
char cmd[100] = {0};
char val[100] = {0};
sprintf(cmd, "i2c_read 0 0xa0 4001 2 1 | grep 0x > /tmp/wibox.type");
system(cmd);
fp = fopen ( "/tmp/wibox.type", "rb" );
if(fp == NULL)
return -1;
fgets(val, 80, fp);
p = val;
while(*p != '\0')
{
if(*p == '\n')
*p = '\0';
p++;
}
fclose(fp);
system("rm /tmp/wibox.type");
//printf("%s\n", val);
p = strstr(val, "0x");
if(p)return (strtol(p+2, NULL, 16));
else return -1;
}
int read_appV(int type, char *appV)
{
FILE *fp = NULL;
if((type == 0x11) || (type == 0x21) )
fp = fopen ( "/root/V9-Wibox.version", "rb" );
else if(type == 0x12)
fp = fopen ( "/root/V9-StreamMonitor.version", "rb" );
if(fp == NULL)
return -1;
fgets(appV, 80, fp);
char *p = appV;
while(*p != '\0')
{
if(*p == '\n')
*p = '\0';
p++;
}
fclose(fp);
//printf("%s\n", val);
return 0;
}
int write_appV(char *version, int type)
{
FILE *fp = NULL;
if((type == 0x11) || (type == 0x21) )
fp = fopen ( "/root/V9-Wibox.version", "w" );
else if(type == 0x12)
fp = fopen ( "/root/V9-StreamMonitor.version", "w" );
if(fp == NULL)
return -1;
fputs(version, fp);
fclose(fp);
//printf("%s\n", val);
return 0;
}
int read_recordV(char *recordV)
{
FILE *fp = NULL;
fp = fopen ( "/root/record.version", "rb" );
if(fp == NULL)
return -1;
fgets(recordV, 100, fp);
fclose(fp);
//printf("%s\n", val);
return 0;
}
int write_recordV(char *version)
{
FILE *fp = NULL;
fp = fopen ( "/root/record.version", "w" );
if(fp == NULL)
return -1;
fputs(version, fp);
fclose(fp);
//printf("%s\n", val);
return 0;
}
int read_rountV(char *rountV)
{
int sockSrv;
struct sockaddr_in servaddr;
int len = 5;
char opcode = 7;
len = htonl(len);
char buf[5] = {0};
char rec_len;
int index=0;
while(1)
{
index++;
sockSrv = socket(AF_INET, SOCK_STREAM, 0);
if (sockSrv == -1)
{
perror("socket error");
return -1;
}
memset(&servaddr, 0, sizeof(servaddr));
servaddr.sin_family = AF_INET;
servaddr.sin_port = htons(ROUTE_PORT);
servaddr.sin_addr.s_addr = inet_addr(ROUTE_IP);
if(connect(sockSrv, (struct sockaddr *)&servaddr, sizeof(servaddr)) == -1)
{
perror("connect error");
//fclose(fp);
close(sockSrv);
if(index>10)
{
return -3;
}
sleep(5);
continue;
}
memcpy(buf, &len, 4);
buf[4] = opcode;
if(send(sockSrv, buf, 5, 0) < 0)
{
perror("send error");
//fclose(fp);
close(sockSrv);
return -2;
}
if(recv(sockSrv, &rec_len, 1, 0) < 0)
{
perror("recv error");
//fclose(fp);
close(sockSrv);
return -2;
}
if(recv(sockSrv, rountV, 10, 0) < 0)
{
perror("recv error");
//fclose(fp);
close(sockSrv);
return -2;
}
close(sockSrv);
return 0;
//printf("rountV:%s\n", rountV);
}
return 0;
}
int read_bsV(char *bsV)
{
FILE *fp = NULL;
system("cat "BS_VERSION_FILE" |grep wibox.version|awk '{print $NF}' > "TMP_FILE"");
fp = fopen (TMP_FILE, "rb" );
if(fp == NULL)
return -1;
fgets(bsV, 100, fp);
fclose(fp);
//printf("%s\n", val);
return 0;
}
void read_version()
{
int name_flag = 1;
char version[100] = {0};
memset(&devinfo, 0, sizeof(devinfo));
name_flag = read_name(version);
if(name_flag == 1)
{
sprintf(devinfo.name, "%s", "910");
}
else if(name_flag == 2)
{
sprintf(devinfo.name, "%s", "920");
}
else
{
printf("read name error\n");
}
if(name_flag == 1)
devinfo.type = 0x21;
else if(name_flag == 2)
{
devinfo.type = read_type();
}
sprintf(devinfo.kernelV, "%s", version);
memset(version, 0, sizeof(version));
read_appV(devinfo.type, version);
sprintf(devinfo.appV, "%s", version);
memset(version, 0, sizeof(version));
read_recordV(version);
sprintf(devinfo.recordV, "%s", version);
memset(version, 0, sizeof(version));
read_bsV(version);
sprintf(devinfo.bsV, "%s", version);
memset(version, 0, sizeof(version));
if(devinfo.type == 0x21)
{
read_rountV(version);
sprintf(devinfo.routeV, "%s", version);
}
}
int main()
{
int err=0;
pthread_t tDev;
setbuf(stdout, NULL);
setbuf(stderr, NULL);
printf("wibox_batch_up version:1.0.0.1\n");
printf("start wibox_batch_up\n");
mk_updates_dir();
read_version();
err=pthread_create(&tDev,NULL,send_devinfo,NULL);
if(err!=0)
{
printf("Create send_devinfo error: %s\n",strerror(errno));
return 0;
}
err=pthread_create(&tDev,NULL,update_thread,NULL);
if(err!=0)
{
printf("Create send_devinfo error: %s\n",strerror(errno));
return 0;
}
while(1)
{
}
return 0;
}
<file_sep>// V9_BATCH_UPDlg.h : 头文件
//
#pragma once
#include "afxcmn.h"
#define THREAD_MAX 8
#define BOARD_MAX 100
enum update_status
{
UP_START,
UP_TRANS_SUC = 10,
UP_TRANS_ERR,
UP_MD5_SUC = 20,
UP_MD5_ERR,
UP_UPDATE_SUC = 30,
UP_UPDATE_ERR
};
struct DEVINFO
{
char name[48];
int type;
char routeV[16];
char kernelV[16];
char appV[16];
char recordV[16];
char bsV[16];
char str1[16];
char str2[16];
};
struct DEVINFOLIST
{
int index;
DEVINFOLIST * pNext;
char ip[20];
char name[48];
int type;
char routeV[16];
char kernelV[16];
char appV[16];
char recordV[16];
char bsV[16];
char str1[16];
char str2[16];
};
struct UPDATEFILE
{
char route[100];
char kernel[100];
char app[100];
char record[100];
char bs[100];
};
// CV9_BATCH_UPDlg 对话框
class CV9_BATCH_UPDlg : public CDialog
{
// 构造
public:
CV9_BATCH_UPDlg(CWnd* pParent = NULL); // 标准构造函数
// 对话框数据
enum { IDD = IDD_V9_BATCH_UP_DIALOG };
protected:
virtual void DoDataExchange(CDataExchange* pDX); // DDX/DDV 支持
// 实现
protected:
HICON m_hIcon;
DEVINFOLIST *m_pHost;
DEVINFOLIST *m_pHostUpdate;
UPDATEFILE updatefile;
// 生成的消息映射函数
virtual BOOL OnInitDialog();
afx_msg void OnSysCommand(UINT nID, LPARAM lParam);
afx_msg void OnPaint();
afx_msg HCURSOR OnQueryDragIcon();
DECLARE_MESSAGE_MAP()
public:
CListCtrl m_listboard;
CListCtrl m_updateboard;
int update_num;
int status[BOARD_MAX];
afx_msg void OnBnClickedRadio1();
afx_msg void OnBnClickedRadio2();
BOOL AddHost(const char * ip, struct DEVINFO *info);
BOOL up_status(const char *ip, int status);
BOOL AddBoardUpdate(int nIndex);
BOOL DelBoardUpdate (const char *ip);
int insertHost(DEVINFOLIST *pos, const char *ip, struct DEVINFO *info);
int insertUpdateHost(DEVINFOLIST *pos, DEVINFOLIST *info);
int delUpdateHost(DEVINFOLIST *pre, DEVINFOLIST *pos);
int listboard();
int listUpdateboard();
int FindUpdates();
int tcp_update();
int ftp_update();
int tcp_send(int num);
int tar_gz();
int count_md5(char *md5);
int addfile(char *files, const char *file);
int updateflag;
char m_file_cvs[100];
DEVINFOLIST * CV9_BATCH_UPDlg::next_update(DEVINFOLIST *p ,int num);
DEVINFOLIST * CV9_BATCH_UPDlg::first_update(DEVINFOLIST *p ,int num);
int update_rec_flag(int num, const char *ip, int index);
int updatefunc();
int CProgressCtrlInit(int range);
int CProgressCtrlUpdate(int num, const char *info);
int count_update_num();
afx_msg void OnBnClickedButton1();
afx_msg void OnBnClickedButton2();
afx_msg void OnBnClickedButton3();
afx_msg void OnBnClickedOk();
};
<file_sep>#include "stdafx.h"
#include "openssl/md5.h"
enum update_status
{
UP_START,
UP_TRANS_SUC = 10,
UP_TRANS_ERR,
UP_MD5_SUC = 20,
UP_MD5_ERR,
UP_UPDATE_SUC = 30,
UP_UPDATE_ERR
};
struct DEVINFO
{
char name[48];
int type;
char routeV[16];
char kernelV[16];
char appV[16];
char recordV[16];
char bsV[16];
char str1[16];
char str2[16];
};
struct DEVINFOLIST
{
int index;
DEVINFOLIST * pNext;
char ip[20];
char name[48];
int type;
char routeV[16];
char kernelV[16];
char appV[16];
char recordV[16];
char bsV[16];
char str1[16];
char str2[16];
};
int file_md5(const char *file, char *md5)
{
CString str;
FILE *fp = fopen(file, "rb");
if(fp == NULL)
{
str = "无法打开升级文件";
AfxMessageBox(str);
return -1;
}
MD5_CTX ctx;
MD5_Init (&ctx);
unsigned char digest[16] = {0};
int len;
unsigned char buffer[1024] = {0};
while ((len = fread (buffer, 1, 1024, fp)) > 0)
{
MD5_Update (&ctx, buffer, len);
}
MD5_Final (digest, &ctx);
fclose(fp);
int i = 0;
char tmp[3] = {0};
for(i = 0; i < 16; i++ )
{
sprintf(tmp,"%02x", digest[i]); // sprintf并不安全
if(md5 == NULL)
{
str = "参数md5不能为NULL";
AfxMessageBox(str);
return -1;
}
strcat(md5, tmp); // strcat亦不是什么好东西
}
fclose(fp);
return 0;
}
int tar_gzip(const char *files, const char *tgz)
{
//char cmd[200] = {0};
char name_tar[100] = {0};
char name_tgz[100] = {0};
//int ret1, ret2;
sprintf(name_tar, "%s.tar", tgz);
sprintf(name_tgz, "%s.gz", name_tar);
//sprintf(cmd, "cmd /c, 7z a -ttar %s %s ", name_tar, file);
//ret1 = WinExec(cmd,SW_HIDE);
////Sleep(1000);
//sprintf(cmd, "cmd /c, 7z a -tgzip %s %s ", name_tgz, name_tar);
//ret2 = WinExec(cmd,SW_HIDE);
//sprintf(cmd, "%d %d", ret1, ret2);
//CString str;
//str = cmd;
//AfxMessageBox(str);
char strCommand[1000] = {0};
STARTUPINFOA si = {sizeof(si)};
si.cb = sizeof(STARTUPINFOA);
si.lpReserved = NULL;
si.lpDesktop = NULL;
si.lpTitle = NULL;
si.dwFlags = STARTF_USESHOWWINDOW;//隐藏窗口
si.wShowWindow = SW_HIDE;
si.cbReserved2 = NULL;
si.lpReserved2 = NULL;
PROCESS_INFORMATION pi;
STARTUPINFOA si2 = {sizeof(si2)};
si2.cb = sizeof(STARTUPINFOA);
si2.lpReserved = NULL;
si2.lpDesktop = NULL;
si2.lpTitle = NULL;
si2.dwFlags = STARTF_USESHOWWINDOW;//隐藏窗口
si2.wShowWindow = SW_HIDE;
si2.cbReserved2 = NULL;
si2.lpReserved2 = NULL;
PROCESS_INFORMATION pi2;
DeleteFile(name_tar);
DeleteFile(name_tgz);
//Sleep(10000);
sprintf(strCommand, "7z a -ttar %s %s ", name_tar, files);
//AfxMessageBox(strCommand);
BOOL bRet = CreateProcessA(NULL,strCommand,NULL,NULL,FALSE,0,NULL,NULL,&si,&pi);
if (bRet)
{
//等待运行完成
if (!WaitForSingleObject(pi.hProcess,INFINITE))
{
memset(strCommand, 0, sizeof(strCommand));
sprintf(strCommand, "7z a -tgzip %s %s ", name_tgz, name_tar);
//Sleep(10000);
//AfxMessageBox(strCommand);
bRet = CreateProcessA(NULL,strCommand,NULL,NULL,FALSE,0,NULL,NULL,&si2,&pi2);
if(!bRet)
{
AfxMessageBox("压缩文件出错");
return -4;
}
if (!WaitForSingleObject(pi2.hProcess,INFINITE))
{
}
else
{
AfxMessageBox("压缩文件指令出错");
return -3;
}
//Sleep(10000);
}
else
{
AfxMessageBox("打包文件指令错误");
return -2;
}
}
else
{
AfxMessageBox("打包文件出错");
return -1;
}
return 0;
}
unsigned long long file_len(const char *file)
{
unsigned long long len;
WIN32_FIND_DATA FindData;
HANDLE hFind = FindFirstFile(file, &FindData );
len = ((unsigned long long)FindData.nFileSizeHigh << 32) + FindData.nFileSizeLow;
FindClose(hFind);
return len;
}
int send_pack(SOCKET s, const char *buf, int len, int flags)
{
int times = 0;
int ret;
CString str;
//str.Format ("len:%u %x %x", len, buf[0], buf[1]);
//AfxMessageBox(str);
while((ret = send(s, buf, len, flags)) < 0)
{
//str.Format ("ret:%u", ret);
//AfxMessageBox(str);
if(times > 3)
{
break;
}
times++;
}
return ret;
}
BOOL CreateCSV (char *file)
{
CString str;
CTime tm;
tm = CTime::GetCurrentTime ();
str = tm.Format ("LOG_%Y%m%d_%H%M%S.csv");
strcpy (file, str);
FILE *fp = fopen (file, "w");
if(fp == NULL)
{
return FALSE;
}
char str2[256];
sprintf (str2, "%s,%s,%s,%s,%s\n", "序号","板卡IP", "板卡名", "板卡类型", "更新状态");
//fputs(str,fp);
fwrite (str2, strlen (str2), 1, fp);
fclose (fp);
return TRUE;
}
BOOL AddToCSV (char *file, struct DEVINFOLIST *host, int *status)
{
FILE *fp = fopen (file, "a");
if (fp)
{
char str[256];
int i = 0;
while(host != NULL)
{
if(status[i] == UP_UPDATE_SUC)
sprintf (str, "%d,%s,%s,0x%x,%s\n", i+1, host->ip, host->name, host->type, "success");
else
sprintf (str, "%d,%s,%s,0x%x,%s\n", i+1, host->ip, host->name, host->type, "error");
//fputs(str,fp);
fwrite (str, strlen (str), 1, fp);
host = host->pNext;
i++;
}
fclose (fp);
}
else
{
return FALSE;
}
return TRUE;
}
| d61b8de580e6b7c9bfb626d1d66a1b6c8291d2fc | [
"Makefile",
"C",
"Text",
"C++"
] | 7 | Text | flyhzgq/V9_BATCH_UP | a8aaf80ef54e227dd039ddc320416fac0c2fc2d1 | 1b1620872e4b432a13fb8349e18857b6c586a87b | |
refs/heads/master | <file_sep>__author__ = '<NAME>, <NAME>'
__copyright__ = 'Approved for public release: AFRL DSRC Case Number 88ABW-2016-0260'
__version__ = '0.5'
__maintainer__ = '<NAME>'
__email__ = '<EMAIL>; <EMAIL>'
__date__ = '9/14/15'
import os
import sys
import six
import glob
import shutil
import string
import logging
import datetime
import traceback
from monty.serialization import loadfn
from pymatgen import Structure
from pymatgen.io.vasp.inputs import Kpoints
from pymatgen.io.vasp.sets import MinimalVaspInputSet
from fireworks import Firework, FireTaskBase, FWAction, explicit_serialize, Workflow, LaunchPad
from custodian import Custodian
from custodian.vasp.jobs import VaspJob
from custodian_afrl.vasp.validators import VasprunXMLValidator
logger = logging.getLogger(__name__)
MODULE_DIR = os.path.dirname(os.path.abspath(__file__))
def load_class(mod, name):
mod = __import__(mod, globals(), locals(), [name], 0)
return getattr(mod, name)
class VaspInputInterface:
"""
This is an interface class to WriteVaspInputTask(FireTaskBase).
Required Params:
-s (obj): Pymatgen Structure Object used to for
creating VASP input files
Optional Params:
-vis (Vasp Input Set): Input Set from pymatgen.io.vasp.sets to use
for default input parameters to VASP
-isp (Input Set param): Custom VASP input parameters to overwrite the
default parameters from the VASP Input Set file.
-custom_params ({dict}): Used to pass custom KPOINT settings for
a VASP calculation.
-config_file (str): Location of a configuration file used to overwrite
VASP input set parameters. If none is given,
it will check for vasp_interface_defaults.yaml in
the user's HOME directory.
"""
def __init__(self,s=None,vis='MinimalVaspInputSet',isp={}, custom_params={}, config_file=None):
# Set ignore list for ignoring certain __dict__ items in member functions
self.__dict__['ignore_list'] = ['input','custom_settings',
'user_incar','vasp_is','ignore_list', 'input_set_params',
'kpts_params', 'params']
if config_file:
config_dict = loadfn(config_file)
elif os.path.exists(os.path.join(os.environ['HOME'], 'vasp_interface_defaults.yaml')):
config_dict = loadfn(os.path.join(os.environ['HOME'], 'vasp_interface_defaults.yaml'))
else:
config_dict = {}
self.__dict__['vasp_is'] = vis
self.__dict__['user_incar'] = isp
self.__dict__['custom_settings'] = custom_params
print("\n*********************************************\n"
"Configuration File not supplied and\n"
"${HOME}/vasp_interface_defaults.yaml does\n"
"not exist. You can copy this file from\n"
"pymatgen/io/vasp/InterfaceExamples/test_files/\n"
"vasp_interface_defaults.yaml and modify it \n"
"for your project's needs.\n"
"*********************************************\n\n"
"Will continue with MinimalVaspInputSet parameters.\n")
if config_dict:
self.__dict__['vasp_is'] = config_dict.get('VASP_INPUT_SET', 'MinimalVaspInputSet')
self.__dict__['user_incar'] = {} if config_dict['INPUT_SET_PARAMS']['user_incar_settings'] == None else config_dict['INPUT_SET_PARAMS']
self.__dict__['custom_settings'] = config_dict.get('CUSTOM_PARAMS', {})
if isp:
self.user_incar['user_incar_settings'].update(isp)
if custom_params:
self.custom_settings['user_kpts_settings'].update(custom_params)
self.__dict__['input']=WriteVaspInputTask(structure=s, vasp_input_set=self.vasp_is,
input_set_params=self.user_incar, custom_params=self.custom_settings)
self.__dict__['params'] = [u'NELMDL', u'LMUSIC', u'WC', u'LCHIMAG', u'INIWAV', u'APACO',
u'IBRION', u'ICORELEVEL', u'MODEL_GW', u'IRESTART', u'MAGMOM',
u'PARAM2', u'POMASS', u'LRPA', u'ADDGRID', u'OFIELD_KAPPA',
u'MAXMIX', u'EMAX', u'LFXHEG', u'LBERRY', u'ENAUG', u'LHFONE',
u'ISYM', u'NREBOOT', u'MODEL_ALPHA', u'AEXX', u'LTCTE',
u'IDIOT', u'ENINI', u'LELF', u'HFALPHA', u'NBANDS', u'LMAXPAW',
u'LSPIRAL', u'ISMEAR', u'ENCUTGWSOFT', u'EBREAK', u'NBANDSO',
u'IWAVPR', u'LCHARG', u'WEIMIN', u'NEDOS', u'LCOMPAT',
u'EFIELD', u'LVEL', u'LDAU', u'LMAXMIX', u'NOMEGAR',
u'ODDONLYGW', u'ISPIN', u'EVENONLY', u'BMIX_MAG', u'LUSE_VDW',
u'NMAXFOCKAE', u'SYMPREC', u'LSYMGRAD', u'LVHAR', u'IBSE',
u'LVTOT', u'LGAUGE', u'TEEND', u'AVECCONST', u'MAGDIPOL',
u'ODDONLY', u'ORBITALMAG', u'MCALPHA', u'LZEROZ', u'SIGMA',
u'SAXIS', u'NGZ', u'TURBO', u'NBLOCK', u'TELESCOPE', u'NCORE',
u'NRMM', u'IDIPOL', u'HFSCREENC', u'EVENONLYGW', u'LRHFCALC',
u'EFERMI', u'NBANDSGWLOW', u'LSCAAWARE', u'OMEGATL', u'EDIFF',
u'LEPSILON', u'FOURORBIT', u'DIPOL', u'LTRIPLET', u'LDIPOL',
u'LSORBIT', u'NUPDOWN', u'PRECFOCK', u'HFSCREEN', u'LTHOMAS',
u'LSCALAPACK', u'NBLK', u'NFREE', u'OFIELD_Q6_FAR', u'ENCUT4O',
u'GGA_COMPAT', u'NELECT', u'LMAXMP2', u'LCORR', u'NBANDSV',
u'EXXOEP', u'AMIX', u'TIME', u'LORBIT', u'RWIGS', u'QSPIRAL',
u'NKREDLFX', u'NKREDLFY', u'NKREDLFZ', u'NKREDLF', u'ALGO',
u'IALGO', u'LPARD', u'PSTRESS', u'PARAM3', u'LMODELHF',
u'PARAM1', u'CSHIFT', u'NGYF', u'LWAVE', u'SELFENERGY', u'NPACO',
u'NELMIN', u'LSPECTRAL', u'ENCUTLF', u'EMIN', u'LMAGBLOCH',
u'LSCALU', u'ICHARG', u'NSIM', u'LHARTREE', u'MODEL_EPS0',
u'I_CONSTRAINED_M', u'GGA', u'OMEGAMIN', u'TEBEG', u'DEPER',
u'NMIN', u'DEG_THRESHOLD', u'NLSPLINE', u'LNICSALL', u'MREMOVE',
u'SCALEE', u'INIMIX', u'AMIN', u'NGZF', u'LMETAGGA', u'NPAR',
u'LUSEW', u'MIXPRE', u'MAXMEM', u'ALDAC', u'L2ORDER', u'NELM',
u'OMEGAGRID', u'PREC', u'TAUPAR', u'NWRITE', u'ALDAX', u'EREF',
u'EDIFFG', u'LHFCALC', u'NBANDSGW', u'SHIFTRED', u'LSUBROT',
u'LFXC', u'KSPACING', u'DIM', u'LFOCKAEDFT', u'NUCIND', u'KPAR',
u'OFIELD_Q6_NEAR', u'NKREDX', u'NKREDY', u'NKREDZ', u'KINTER',
u'LASPH', u'VOSKOWN', u'ISIF', u'KGAMMA', u'SMASS', u'ENMAX',
u'LFXCEPS', u'AMIX_MAG', u'OFIELD_K', u'BMIX', u'ISTART',
u'AGGAC', u'NGX', u'LDIAG', u'NGY', u'LOPTICS', u'LASYNC',
u'Zab_VDW', u'AGGAX', u'NSW', u'ENCUTGW', u'ROPT', u'STM',
u'LNABLA', u'NOMEGA', u'SCISSOR', u'POTIM', u'LPLANE', u'LREAL',
u'KBLOCK', u'NGXF', u'DARWINR', u'MAGATOM', u'DARWINV', u'NKRED',
u'LORBITALREAL', u'EPSILON', u'LNONCOLLINEAR', u'OMEGAMAX',
u'SYSTEM', u'IMIX', u'MAGPOS', u'LMAXFOCK', u'OFIELD_A',
u'LMONO', u'ANTIRES', u'LTETE', u'LADDER', u'ENCUT', u'ZVAL']
self.__dict__['kpts_params'] = ['kpts_style', 'kpts', 'kpts_shift']
self.__dict__['input_set_params'] = ['constrain_total_magmom','sort_structure',
'ediff_per_atom','potcar_functional','force_gamma','reduce_structure']
#Set dictionaries in case they do not exsist
try:
self.input['input_set_params']['user_incar_settings']
except:
self.input['input_set_params']['user_incar_settings'] = {}
try:
self.input['custom_params']['user_kpts_settings']
except:
self.input['custom_params']['user_kpts_settings'] = {}
def __setattr__(self, key, val):
'''
Automatically adds Class Object Attributes directly to their
appropriate dictionary for the WriteVaspInputTask
Example:
This will put NEDOS=2048 into the WriteVaspInputTask['input_set_params']\
['user_incar_settings']['NEDOS']=2048
input = VaspInputInterface(s=s)
input.NEDOS=2048
This will put KPTS=[12,12,12] into WriteVaspInputTask['custom_params']\
['user_kpts_settings']['kpts']=[12,12,12]
input.kpts=[12,12,12]
'''
self.proc_key_val(key.strip(), val.strip()) if isinstance(
val, six.string_types) else self.proc_key_val(key.strip(),val)
def __setitem__(self, key, val):
'''
Automatically adds Class Dictionary Entries directly to their
appropriate dictionary for WriteVaspInputTask.
Example:
This will put NEDOS=2048 into the WriteVaspInputTask['input_set_params']\
['user_incar_settings']['NEDOS']=2048
input = VaspInputInterface(s=s)
input['NEDOS']=2048
'''
self.proc_key_val(key.strip(), val.strip()) if isinstance(
val, six.string_types) else self.proc_key_val(key.strip(),val)
def proc_key_val(self, key, val):
'''
Internal method for sorting Class attributes and Class Dictionary
entries into their appropriate WriteVaspInputTask Dictionary
'''
incar_dict = self.input.get('input_set_params',
{}).get('user_incar_settings', {})
kpts_dict = self.input.get('custom_params',
{}).get('user_kpts_settings', {})
if key not in self.params and key not in self.kpts_params and \
key not in self.ignore_list and key not in self.input_set_params:
print "Parameter '{}' not found in any parameter list".format(key)
print "Please check spelling and try again."
print("Adding {} to base <VaspInputInterface Obj> Attribute\n\n".format(key))
if key in self.params:
incar_dict.update({key: val})
elif key in self.kpts_params:
kpts_dict.update({key: val})
elif key in self.input_set_params:
self.input['input_set_params'].update({key:val})
else:
self.__dict__.update({key:val})
def write_input(self,dir='.'):
self.input.write_input_task(dir)
def get_nelect(self):
return self.input.get_nelect_task()
def add_to_allowed_list(self,parameter):
self.__dict__['params'].append(parameter)
class VaspFirework():
"""
This is an interface to Fireworks for VaspInputInterface workflows
Required Params:
-vasp_task (obj): FireTask Object used to create a Firework.
2 FireTasks are automatically created upon constructing
the VaspFirework object from a WriteVaspInputTask:
[<WriteVaspInputTask()>, <RunCustodianTask()>].
Optional Params:
-name (str): Name given to the Firework
"""
def __init__(self,vasp_task=None,name='vaspfw',handlers=None, handler_params=None, config_file=None):
self.name = name
self.handlers=handlers if handlers else []
self.handler_params=handler_params if handler_params else {}
if config_file:
config_dict = loadfn(config_file)
elif os.path.exists(os.path.join(os.environ['HOME'], 'vasp_interface_defaults.yaml')):
config_dict = loadfn(os.path.join(os.environ['HOME'], 'vasp_interface_defaults.yaml'))
else:
config_dict = {}
if config_dict:
self.custodian_opts = config_dict.get('CUSTODIAN_PARAMS', {})
if self.custodian_opts.get('handlers', []):
self.handlers.extend(self.custodian_opts.get('handlers', []))
self.handler_params.update(self.custodian_opts.get('handler_params', {}))
self.tasks=[vasp_task.input,RunCustodianTask(handlers=self.handlers,
handler_params=self.handler_params)] if isinstance(vasp_task,
VaspInputInterface) else [vasp_task]
self.Firework=Firework(self.tasks,name=self.name)
# Try to establish connection with Launchpad
try:
self.LaunchPad=LaunchPad.from_file(os.path.join(os.environ["HOME"], ".fireworks", "my_launchpad.yaml"))
except:
self.LaunchPad = None
def add_task(self, task):
'''
Function used to add another FireTask to the Firework. If
given task is a VaspInputInterface Object, it will create the WriteInputTask
as well as add another RunCustodianTask() to the FireTask list.
'''
if isinstance(task, VaspInputInterface):
self.tasks.extend([task.input,
RunCustodianTask(handlers=self.handlers,
handler_params=self.handler_params)])
else:
self.tasks.append(task)
self.Firework=Firework(self.tasks,name=self.name)
def to_file(self,file_name):
self.Firework.to_file(file_name)
def add_fw_to_launchpad(self):
if self.LaunchPad:
self.Workflow=Workflow([self.Firework])
self.LaunchPad.add_wf(self.Workflow)
else:
print("No connection to LaunchPad. \n"
"Use 'to_file(<filename>)' to write a yaml file\n"
"to manually add Firework to LaunchPad later.\n")
def copy_files_from_previous(self, *args, **kwargs):
'''
Function used to automatically insert VASPTransferTask
between WriteVaspInputTask() and RunCustodianTask() in the
FireTask list. This is used to copy files from a previous
VASP Firework Calculation or from a known directory.
Known directories require the full path of the directory.
This function should not be used to copy files from a
previous Firetask within the same Firework.
Parameters:
-mode (str): Mode to use in transferring files:
move, mv, copy, cp, copy2, copytree, copyfile
Default: 'copy'
-ignore_errors (bool): Whether to quit upon error.
Default: True
-dir (str): Directory to transfer files from if not copying
directly from a previous Firework
Default: None
Example:
fw2.copy_files_from_previous('file1', 'file2', ... , 'filen', mode='copy', ignore_errors=False)
Example to copy files from directory other than previous Firework:
fw2.copy_files_from_previous('file1', 'file2', ... , 'filen',
dir='/path/to/dir', mode='copy', ignore_errors=False)
'''
mode = kwargs.get('mode', 'copy')
ignore_errors = kwargs.get('ignore_errors', True)
files = args[0] if isinstance(args[0], list) else [k for k in args]
self.dir = kwargs.get('dir', None)
if self.dir:
self.transfer=VaspTransferTask(files=files, dir=self.dir,
mode=mode, ignore_errors=ignore_errors)
else:
self.transfer=VaspTransferTask(files=files, mode=mode, ignore_errors=ignore_errors)
if isinstance(self.tasks[-1], RunCustodianTask):
self.tasks.pop(-1)
self.add_task(self.transfer)
self.add_task(RunCustodianTask(handlers=self.handlers,
handler_params=self.handler_params))
else:
self.add_task(self.transfer)
def add_handler(self, handler, **kwargs):
'''
Member function to add handler and handler options to
all Fireworks in the VaspFirework.tasks list.
Example (assuming fw1 is the firework object):
fw1.add_handler('WalltimeHandler', wall_time=3600, buffer_time=300)
fw1.add_handler('FrozenJobErrorHandler')
'''
for i,j in enumerate(self.Firework.tasks):
if isinstance(j, RunCustodianTask):
cust_handlers = j.get('handlers', [])
cust_params = j.get('handler_params', {})
if handler not in cust_handlers:
j['handlers'].append(handler)
j['handler_params'].update(kwargs)
self.Firework.spec['_tasks']=[t.to_dict() for t in
self.Firework.tasks] #re-initialize FW.spec
class VaspWorkflow():
"""
A VaspWorkflow encapsulates multiple VaspFirework objects into a Single Workflow.
If the kwarg "dependency" is not set, it will create a Sequential Workflow where the next
Firework in the Workflow will not start before the currently running Firework in the
Workflow completes.
Parameters:
-args (obj): List of VaspFirework objects
-deps_dict {dict}: Specifies the dependency of the VaspInputInterface objects given.
If no dependency is given, Firworks are assumed to be
sequentially dependent.
-name (str): Name to be given to the Workflow
Example:
VaspWorkflow(FW1, FW2, FW3, FW4, deps_dict={FW1: [FW2, FW3], FW2: [FW4], FW3: [FW4]}, name='Example WF')
This will create a Workflow containing the 4 given VaspFirework objects
with a Workflow name of 'Example WF' and the given dependencies.
Dependency Dictionary Explanation:
In the above example, FW2 and FW3 will not start before FW1 is complete.
Likewise, FW4 depends on the completion of FW2 and FW3 before starting.
"""
def __init__(self, *args, **kwargs):
'''
:param args: (VaspFirework objects) objects to create Workflow from. No limit
on the amount of VaspInputInterface objects to be given. Entered as just
comma separated objects passed to class.
:param deps_dict: (dict) specifies the dependency of the VaspInputInterface objects given.
If no dependency is given, Firworks are assumed to be
sequentially dependent.
:param name (str) Name given to Workflow
'''
self.fws = []
self.name = kwargs.get('name', 'Sequential WF')
self.deps_dict = kwargs.get('deps_dict', {})
self.dependency = {}
if self.deps_dict:
for i in self.deps_dict.keys():
fw_deps = []
for j in self.deps_dict[i]:
fw_deps.append(j.Firework)
self.dependency[i.Firework]=fw_deps
self.deps = True if self.dependency else False
for id, fw_task in enumerate(args):
self.fws.append(fw_task.Firework)
if not self.deps and id != 0:
self.dependency[self.fws[id-1]]=[fw_task.Firework]
self.wf = Workflow(self.fws, self.dependency, name=self.name)
# Try to establish connection with Launchpad
try:
self.LaunchPad=LaunchPad.from_file(os.path.join(os.environ["HOME"], ".fireworks", "my_launchpad.yaml"))
except:
self.LaunchPad = None
def add_fw(self, fw_task, deps=None):
self.fws.append(fw_task.Firework)
if deps:
for i in deps.keys():
fw_deps = []
for j in deps[i]:
fw_deps.append(j.Firework)
self.dependency[i.Firework]=fw_deps
else:
id = len(self.fws) - 2
self.dependency[self.fws[id]]=[fw_task.Firework]
self.wf=Workflow(self.fws, self.dependency, name=self.name)
def to_file(self,filename):
self.wf.to_file(filename)
def add_wf_to_launchpad(self):
if self.LaunchPad:
self.LaunchPad.add_wf(self.Workflow)
else:
print("No connection to LaunchPad. \n"
"Use 'to_file(<filename>)' to write a yaml file\n"
"to manually add Workflow to LaunchPad later.\n")
@explicit_serialize
class WriteVaspInputTask(FireTaskBase):
"""
Writes VASP Input files.
Required params:
structure (dict): An input structure in pymatgen's Structure.to_dict
format.
vasp_input_set (str): A string name for the VASP input set. E.g.,
"MPVaspInputSet" or "MITVaspInputSet".
Optional params:
input_set_params (dict): If the input set requires some additional
parameters, specify them using input_set_params. Note that if you
want to change the user_incar_settings, you need to provide
{"input_set_params": {"user_incar_settings": ...}}. This is
because input_set_params caters to not just user_incar_settings,
but all other potential args to the input set.
"""
required_params = ["structure", "vasp_input_set"]
optional_params = ["input_set_params","custom_params"]
def run_task(self, fw_spec):
prev_dir = fw_spec.get('PREV_DIR', None)
self.custom_params = self.get('custom_params', None)
if isinstance(self["structure"], Structure):
s = self["structure"]
elif isinstance(self["structure"], dict):
s = Structure.from_dict(self["structure"])
else:
s = Structure.from_file(os.path.join(prev_dir, self["structure"]))
vis = load_class("pymatgen.io.vasp.sets", self["vasp_input_set"])(
**self.get("input_set_params", {}))
vis.write_input(s, ".")
# Write Custom KPOINTS settings if necessary
ksettings = self.custom_params.get('user_kpts_settings', None) if isinstance(
self.custom_params, dict) else None
if ksettings:
style = ksettings.get('kpts_style', 'Gamma')
kpoints = ksettings.get('kpts', [16,16,16])
shift = ksettings.get('kpts_shift', [0,0,0])
k = Kpoints(kpts=[kpoints], kpts_shift=shift)
k.style = style
k.write_file("KPOINTS")
def write_input_task(self, dir='.'):
self.custom_params = self.get('custom_params', None)
if isinstance(self["structure"], Structure):
s = self["structure"]
elif isinstance(self["structure"], dict):
s = Structure.from_dict(self["structure"])
else:
s = Structure.from_file(self["structure"])
if os.environ.get('VASP_PSP_DIR') == None:
print "VASP_PSP_DIR not set. Checking User's HOME directory for VASP potentials."
if os.path.exists(os.path.join(os.environ.get('HOME'), 'Potentials')):
os.environ['VASP_PSP_DIR'] = os.path.join(os.environ.get('HOME'), 'Potentials')
else:
print "VASP Potentials not found!"
print "Please copy the Potentials Folder"
print "from VASP into your HOME directory"
sys.exit()
vis = load_class("pymatgen.io.vasp.sets", self["vasp_input_set"])(
**self.get("input_set_params", {}))
vis.write_input(s, dir)
# Write Custom KPOINTS settings if necessary
ksettings = self.custom_params.get('user_kpts_settings', None) if isinstance(
self.custom_params, dict) else None
if ksettings:
style = ksettings.get('kpts_style', 'Gamma')
kpoints = ksettings.get('kpts', [16,16,16])
shift = ksettings.get('kpts_shift', [0,0,0])
k = Kpoints(kpts=[kpoints], kpts_shift=shift)
k.style = style
filename = os.path.join(dir, 'KPOINTS')
k.write_file(filename)
print "Wrote VASP input files to '{}'".format(dir)
def get_nelect_task(self):
if isinstance(self["structure"], Structure):
s = self["structure"]
elif isinstance(self["structure"], dict):
s = Structure.from_dict(self["structure"])
else:
s = Structure.from_file(self["structure"])
if os.environ.get('VASP_PSP_DIR') == None:
if os.path.exists(os.path.join(os.environ.get('HOME'), 'Potentials')):
os.environ['VASP_PSP_DIR'] = os.path.join(os.environ.get('HOME'), 'Potentials')
else:
print "VASP_PSP_DIR not set. Check User's HOME directory for VASP potentials."
print "Potentials not found!"
sys.exit()
vis = load_class("pymatgen.io.vasp.sets", self["vasp_input_set"])(
**self.get("input_set_params", {}))
return vis.get_nelect(s)
@explicit_serialize
class VaspTransferTask(FireTaskBase):
"""
A FireTask to Transfer files from a preivous VASP run in the same Workflow.
Required params:
- mode: (str) - move, mv, copy, cp, copy2, copytree, copyfile
- files: [list] - list of files to pull from previous Firework in the Workflow
Optional params:
- ignore_errors (bool) - Whether or not to raise Errors and terminate task.
- dir (str) - Directory from which to copy files from, otherwise PREV_DIR
is used.
"""
required_params = ['mode', 'files']
optional_params = ['ignore_errors', 'dir']
fn_list = {
"move": shutil.move,
"mv": shutil.move,
"copy": shutil.copy,
"cp": shutil.copy,
"copy2": shutil.copy2,
"copytree": shutil.copytree,
"copyfile": shutil.copyfile,
}
def run_task(self, fw_spec):
prev_dir = fw_spec.get('PREV_DIR', self.get('dir', None))
if not prev_dir or not os.path.exists(prev_dir):
raise RuntimeError(
'Source Directory not found or was not specified\n'
'Looked for: {}'.format(prev_dir))
ignore_errors = self.get('ignore_errors', False)
mode = self.get('mode', 'copy')
for f in self['files']:
try:
if '*' in f:
file_list = glob.glob(os.path.join(prev_dir,f))
for src in file_list:
VaspTransferTask.fn_list[mode](src, '.')
else:
src = os.path.join(prev_dir, f)
VaspTransferTask.fn_list[mode](src, '.')
except:
if not ignore_errors:
traceback.print_exc()
raise ValueError(
"There was an error performing operation {} from {} "
"to '.'".format(mode, self["files"]))
@explicit_serialize
class RunCustodianTask(FireTaskBase):
"""
Runs VASP using Custodian.
Optional Params:
handlers ([str]): List of error handler names to use. See custodian
.vasp.handlers for list of applicable handlers. Note that
default args are assumed for all handlers for simplicity. A
special option is "all", which simply uses a set of common
handlers for relaxation jobs.
"""
# Insert way to Add Handler Options and
def run_task(self, fw_spec):
FORMAT = '%(asctime)s %(levelname)s: %(pathname)s\n\t%(module)s.%(funcName)s: %(message)s'
logging.basicConfig(format=FORMAT, level=logging.INFO, filename="run.log")
job = VaspJob(["vasp"],default_vasp_input_set=MinimalVaspInputSet(),auto_npar=False)
#Set up Handlers to be used
handler_param_dict = {'VaspErrorHandler': ['output_filename'],
'AliasingErrorHandler': ['output_filename'],
'MeshSymmetryErrorHandler': ['output_filename', 'output_vasprun'],
'UnconvergedErrorHandler': ['output_filename'],
'MaxForceErrorHandler': ['output_filename', 'max_force_threshold'],
'PotimErrorHandler': ['input_filename',
'output_filename', 'dE_threshold'],
'FrozenJobErrorHandler': ['output_filename', 'timeout'],
'NonConvergingErrorHandler': ['output_filename', 'nionic_steps',
'change_algo'],
'WalltimeHandler': ['wall_time', 'buffer_time',
'electronic_step_stop'],
'CheckpointHandler': ['interval'],
'PositiveEnergyErrorHandler': ['output_filename']}
hnames = self.get('handlers')
handler_params = self.get("handler_params", {})
logging.info("handler names: {}".format(hnames))
handlers = []
for n in hnames:
np = {}
for m in handler_params:
if m in handler_param_dict[n]:
np[m] = handler_params[m]
handlers.append(load_class("custodian.vasp.handlers", n)(np))
c = Custodian(handlers=handlers, validators=[VasprunXMLValidator()], jobs=[job])
output = c.run()
return FWAction(stored_data=output, mod_spec=[{'_set': {'PREV_DIR': os.getcwd()}}])
<file_sep>#!/usr/bin/env python
import os
import sys
import string
import json
import inspect
from pymatgen import Structure
from fireworks import Firework, Workflow, LaunchPad
from pymatgen.io.vasp.interfaces import VaspInputInterface, VaspFirework, VaspWorkflow
# get structure from Crystallographic Information File (CIF)
s = Structure.from_file('./mp-33088_Cr2FeO4.cif')
input=VaspInputInterface(s)
input.NEDOS=2000 # override default or add INCAR parameter
# Dump VASP Input into current directory for inspection
input.write_input()
# Complete definition of Firework Task(s) and add to
# Firework
task=VaspFirework(input)
# Save specification to yaml file for later inspection
# or manual add to launchpad with lpad script
task.to_file("simple_task.yaml")
# Adds single Firework to launchpad database
task.add_fw_to_launchpad()
<file_sep>"""
Created on Aug 7, 2015
"""
__author__ = "<NAME>"
__copyright__ = "Approved for public release: AFRL DSRC Case Number 88ABW-2016-0260"
__version__ = "0.1"
__maintainer__ = "<NAME>"
__email__ = "<EMAIL>"
__date__ = "2016-01-29"
import unittest
import shutil
import pkgutil
import os
if pkgutil.find_loader('fireworks'):
from pymatgen.io.vasp.interfaces import VaspInputInterface, VaspFirework, VaspWorkflow, VaspTransferTask
from fireworks import ScriptTask
from pymatgen import Structure
MODULE_DIR = os.path.dirname(os.path.abspath(__file__))
test_dir = os.path.join(MODULE_DIR, '..', 'InterfaceExamples', 'test_files')
@unittest.skipUnless(1 if pkgutil.find_loader("fireworks") else 0, "Requires Fireworks")
class VaspInputInterfaceTest(unittest.TestCase):
def setUp(self):
self.maxDiff=None
self.mol_dict = {u'@class': 'Structure',
u'@module': 'pymatgen.core.structure',
u'lattice': {u'a': 3.09681658,
u'alpha': 60.00000352,
u'b': 3.09681633,
u'beta': 60.000006230000004,
u'c': 3.096817,
u'gamma': 60.00000143999999,
u'matrix': [[2.6819219975054747, 0.0, 1.5484079983838697],
[0.8939740004171242, 2.5285400003050134, 1.5484080002345615],
[0.0, 0.0, 3.096817]],
u'volume': 21.00059082235557},
u'sites': [{u'abc': [0.25, 0.25, 0.25],
u'species': [{u'element': u'Si', u'occu': 1.0}]},
{u'abc': [0.0, 0.0, 0.0],
u'species': [{u'element': u'C', u'occu': 1.0}]}]}
self.mol_file = os.path.join(test_dir, 'SiC_0.cif')
self.config_file = os.path.join(test_dir, 'vasp_interface_defaults.yaml')
self.isp = {'ALGO': 'Fast', 'LOPTICS':False}
self.custom_params = {'kpts': [8,8,8], 'kpts_shift':[1,0,0]}
self.gdic = {u'_fw_name': '{{pymatgen.io.vasp.interfaces.WriteVaspInputTask}}',
'custom_params': {'user_kpts_settings': {'kpts': [6, 6, 6],
'kpts_shift': [0, 0, 0],
'kpts_style': 'Gamma'}},
'input_set_params': {'user_incar_settings': {'ALGO': 'Normal',
'CSHIFT': 0.1,
'LOPTICS': '.TRUE.',
'NEDOS': 4096,
'SIGMA': 0.01}},
'structure': {'@class': 'Structure',
'@module': 'pymatgen.core.structure',
'lattice': {'a': 3.09681658,
'alpha': 60.00000352,
'b': 3.09681633,
'beta': 60.000006230000004,
'c': 3.096817,
'gamma': 60.00000143999999,
'matrix': [[2.6819219975054747, 0.0, 1.5484079983838697],
[0.8939740004171242, 2.5285400003050134, 1.5484080002345615],
[0.0, 0.0, 3.096817]],
'volume': 21.00059082235557},
'sites': [{'abc': [0.25, 0.25, 0.25],
'species': [{'element': 'Si', 'occu': 1.0}]},
{'abc': [0.0, 0.0, 0.0], 'species': [{'element': 'C', 'occu': 1.0}]}]},
'vasp_input_set': 'MinimalVaspInputSet'}
def tearDown(self):
pass
def test_input_from_dict(self):
input = VaspInputInterface(s=self.mol_dict, config_file=self.config_file)
inp_dic = input.input.as_dict()
self.assertDictEqual(inp_dic, self.gdic, msg=None)
def test_input_from_file(self):
input = VaspInputInterface(s=self.mol_file, config_file=self.config_file)
inp_dic = input.input.as_dict()
cdic = {u'_fw_name': '{{pymatgen.io.vasp.interfaces.WriteVaspInputTask}}',
'custom_params': {'user_kpts_settings': {'kpts': [6, 6, 6],
'kpts_shift': [0, 0, 0],
'kpts_style': 'Gamma'}},
'input_set_params': {'user_incar_settings': {'ALGO': 'Normal',
'CSHIFT': 0.1,
'LOPTICS': '.TRUE.',
'NEDOS': 4096,
'SIGMA': 0.01}},
'structure': self.mol_file,
'vasp_input_set': 'MinimalVaspInputSet'}
self.assertDictEqual(inp_dic, cdic, msg=None)
def test_input_from_structure(self):
s = Structure.from_file(self.mol_file)
input = VaspInputInterface(s=s, config_file=self.config_file)
inp_dic = input.input.as_dict()
self.assertDictEqual(inp_dic, self.gdic, msg=None)
def test_custom_incar_and_kpoints(self):
input = VaspInputInterface(s=self.mol_dict, isp=self.isp,
custom_params=self.custom_params,
config_file=self.config_file)
inp_dic = input.input.as_dict()
cdic = {u'_fw_name': '{{pymatgen.io.vasp.interfaces.WriteVaspInputTask}}',
'custom_params': {'user_kpts_settings': {'kpts': [8, 8, 8],
'kpts_shift': [1, 0, 0],
'kpts_style': 'Gamma'}},
'input_set_params': {'user_incar_settings': {'ALGO': 'Fast',
'CSHIFT': 0.1,
'LOPTICS': False,
'NEDOS': 4096,
'SIGMA': 0.01}},
'structure': {'@class': 'Structure',
'@module': 'pymatgen.core.structure',
'lattice': {'a': 3.09681658,
'alpha': 60.00000352,
'b': 3.09681633,
'beta': 60.000006230000004,
'c': 3.096817,
'gamma': 60.00000143999999,
'matrix': [[2.6819219975054747, 0.0, 1.5484079983838697],
[0.8939740004171242, 2.5285400003050134, 1.5484080002345615],
[0.0, 0.0, 3.096817]],
'volume': 21.00059082235557},
'sites': [{'abc': [0.25, 0.25, 0.25],
'species': [{'element': 'Si', 'occu': 1.0}]},
{'abc': [0.0, 0.0, 0.0], 'species': [{'element': 'C', 'occu': 1.0}]}]},
'vasp_input_set': 'MinimalVaspInputSet'}
self.assertDictEqual(inp_dic, cdic, msg=None)
def test_user_friendly_custom_incar(self):
input = VaspInputInterface(s=self.mol_file, config_file=self.config_file)
input.LOPTICS=False
input.ALGO='Exact'
input.EDIFF=1e-05
input.kpts=[8,8,8]
input.kpts_shift=[1,0,0]
inp_dic = input.input.as_dict()
cdic = {u'_fw_name': '{{pymatgen.io.vasp.interfaces.WriteVaspInputTask}}',
'custom_params': {'user_kpts_settings': {'kpts': [8, 8, 8],
'kpts_shift': [1, 0, 0],
'kpts_style': 'Gamma'}},
'input_set_params': {'user_incar_settings': {'ALGO': 'Exact',
'CSHIFT': 0.1,
'EDIFF': 1e-05,
'LOPTICS': False,
'NEDOS': 4096,
'SIGMA': 0.01}},
'structure': self.mol_file,
'vasp_input_set': 'MinimalVaspInputSet'}
self.assertDictEqual(inp_dic, cdic, msg=None)
def test_get_nelect_from_interface(self):
input = VaspInputInterface(s=self.mol_file, config_file=self.config_file)
nelect = input.get_nelect()
self.assertEqual(nelect, 8.0, msg=None)
@unittest.skipUnless(1 if pkgutil.find_loader("fireworks") else 0, "Requires Fireworks")
class VaspFireworkTest(unittest.TestCase):
def setUp(self):
self.mol_file = 'SiC_0.cif'
self.config_file = os.path.join(test_dir, 'vasp_interface_defaults.yaml')
self.input = VaspInputInterface(s=self.mol_file, config_file=self.config_file)
self.misc_task = ScriptTask.from_str("echo 'Hello World!'")
def tearDown(self):
pass
def test_add_task_VaspInputInterface(self):
fw = VaspFirework(self.input, config_file=self.config_file)
fw.add_task(self.input)
fw_dic = fw.Firework.as_dict()
for i in ['created_on', 'updated_on', 'fw_id']:
del fw_dic[i]
gdic = {'name': 'vaspfw',
'spec': {'_tasks': [{'_fw_name': '{{pymatgen.io.vasp.interfaces.WriteVaspInputTask}}',
'custom_params': {'user_kpts_settings': {'kpts': [6, 6, 6],
'kpts_shift': [0, 0, 0],
'kpts_style': 'Gamma'}},
'input_set_params': {'user_incar_settings': {'ALGO': 'Normal',
'CSHIFT': 0.1,
'LOPTICS': '.TRUE.',
'NEDOS': 4096,
'SIGMA': 0.01}},
'structure': 'SiC_0.cif',
'vasp_input_set': 'MinimalVaspInputSet'},
{'_fw_name': '{{pymatgen.io.vasp.interfaces.RunCustodianTask}}',
'handler_params': {},
'handlers': []},
{'_fw_name': '{{pymatgen.io.vasp.interfaces.WriteVaspInputTask}}',
'custom_params': {'user_kpts_settings': {'kpts': [6, 6, 6],
'kpts_shift': [0, 0, 0],
'kpts_style': 'Gamma'}},
'input_set_params': {'user_incar_settings': {'ALGO': 'Normal',
'CSHIFT': 0.1,
'LOPTICS': '.TRUE.',
'NEDOS': 4096,
'SIGMA': 0.01}},
'structure': 'SiC_0.cif',
'vasp_input_set': 'MinimalVaspInputSet'},
{'_fw_name': '{{pymatgen.io.vasp.interfaces.RunCustodianTask}}',
'handler_params': {},
'handlers': []}]}}
self.assertDictEqual(fw_dic, gdic, msg=None)
def test_add_task_misc_task(self):
fw = VaspFirework(self.input, config_file=self.config_file)
fw.add_task(self.misc_task)
fw_dic = fw.Firework.as_dict()
for i in ['created_on', 'updated_on', 'fw_id']:
del fw_dic[i]
gdic = {'name': 'vaspfw',
'spec': {'_tasks': [{'_fw_name': '{{pymatgen.io.vasp.interfaces.WriteVaspInputTask}}',
'custom_params': {'user_kpts_settings': {'kpts': [6, 6, 6],
'kpts_shift': [0, 0, 0],
'kpts_style': 'Gamma'}},
'input_set_params': {'user_incar_settings': {'ALGO': 'Normal',
'CSHIFT': 0.1,
'LOPTICS': '.TRUE.',
'NEDOS': 4096,
'SIGMA': 0.01}},
'structure': 'SiC_0.cif',
'vasp_input_set': 'MinimalVaspInputSet'},
{'_fw_name': '{{pymatgen.io.vasp.interfaces.RunCustodianTask}}',
'handler_params': {},
'handlers': []},
{'_fw_name': 'ScriptTask',
'script': ["echo 'Hello World!'"],
'use_shell': True}]}}
self.assertDictEqual(fw_dic, gdic, msg=None)
def test_copy_from_previous(self):
fw = VaspFirework(self.input, config_file=self.config_file)
fw.copy_files_from_previous('WAVECAR', 'WAVEDER', mode='copy', ignore_errors=True)
fw_dic = fw.Firework.as_dict()
for i in ['created_on', 'updated_on', 'fw_id']:
del fw_dic[i]
gdic = {'name': 'vaspfw',
'spec': {'_tasks': [{'_fw_name': '{{pymatgen.io.vasp.interfaces.WriteVaspInputTask}}',
'custom_params': {'user_kpts_settings': {'kpts': [6, 6, 6],
'kpts_shift': [0, 0, 0],
'kpts_style': 'Gamma'}},
'input_set_params': {'user_incar_settings': {'ALGO': 'Normal',
'CSHIFT': 0.1,
'LOPTICS': '.TRUE.',
'NEDOS': 4096,
'SIGMA': 0.01}},
'structure': 'SiC_0.cif',
'vasp_input_set': 'MinimalVaspInputSet'},
{'_fw_name': '{{pymatgen.io.vasp.interfaces.VaspTransferTask}}',
'files': ['WAVECAR', 'WAVEDER'],
'ignore_errors': True,
'mode': 'copy'},
{'_fw_name': '{{pymatgen.io.vasp.interfaces.RunCustodianTask}}',
'handler_params': {},
'handlers': []}]}}
self.assertDictEqual(fw_dic, gdic, msg=None)
def test_copy_from_dir(self):
out_name = 'FW_copy_from_dir.yaml'
fw = VaspFirework(self.input, config_file=self.config_file)
fw.copy_files_from_previous('WAVECAR', 'WAVEDER', mode='copy',
dir='../../test_files/VASP_INTERFACES_TEST_FILES',
ignore_errors=True)
fw_dic = fw.Firework.as_dict()
for i in ['created_on', 'updated_on', 'fw_id']:
del fw_dic[i]
gdic = {'name': 'vaspfw',
'spec': {'_tasks': [{'_fw_name': '{{pymatgen.io.vasp.interfaces.WriteVaspInputTask}}',
'custom_params': {'user_kpts_settings': {'kpts': [6, 6, 6],
'kpts_shift': [0, 0, 0],
'kpts_style': 'Gamma'}},
'input_set_params': {'user_incar_settings': {'ALGO': 'Normal',
'CSHIFT': 0.1,
'LOPTICS': '.TRUE.',
'NEDOS': 4096,
'SIGMA': 0.01}},
'structure': 'SiC_0.cif',
'vasp_input_set': 'MinimalVaspInputSet'},
{'_fw_name': '{{pymatgen.io.vasp.interfaces.VaspTransferTask}}',
'dir': '../../test_files/VASP_INTERFACES_TEST_FILES',
'files': ['WAVECAR', 'WAVEDER'],
'ignore_errors': True,
'mode': 'copy'},
{'_fw_name': '{{pymatgen.io.vasp.interfaces.RunCustodianTask}}',
'handler_params': {},
'handlers': []}]}}
self.assertDictEqual(fw_dic, gdic, msg=None)
def test_combo_misc_add_task(self):
out_name = 'FW_combo_add_tasks.yaml'
fw = VaspFirework(self.input, config_file=self.config_file)
fw.add_task(self.misc_task)
fw.add_task(self.input)
fw_dic = fw.Firework.as_dict()
for i in ['created_on', 'updated_on', 'fw_id']:
del fw_dic[i]
gdic = {'name': 'vaspfw',
'spec': {'_tasks': [{'_fw_name': '{{pymatgen.io.vasp.interfaces.WriteVaspInputTask}}',
'custom_params': {'user_kpts_settings': {'kpts': [6, 6, 6],
'kpts_shift': [0, 0, 0],
'kpts_style': 'Gamma'}},
'input_set_params': {'user_incar_settings': {'ALGO': 'Normal',
'CSHIFT': 0.1,
'LOPTICS': '.TRUE.',
'NEDOS': 4096,
'SIGMA': 0.01}},
'structure': 'SiC_0.cif',
'vasp_input_set': 'MinimalVaspInputSet'},
{'_fw_name': '{{pymatgen.io.vasp.interfaces.RunCustodianTask}}',
'handler_params': {},
'handlers': []},
{'_fw_name': 'ScriptTask',
'script': ["echo 'Hello World!'"],
'use_shell': True},
{'_fw_name': '{{pymatgen.io.vasp.interfaces.WriteVaspInputTask}}',
'custom_params': {'user_kpts_settings': {'kpts': [6, 6, 6],
'kpts_shift': [0, 0, 0],
'kpts_style': 'Gamma'}},
'input_set_params': {'user_incar_settings': {'ALGO': 'Normal',
'CSHIFT': 0.1,
'LOPTICS': '.TRUE.',
'NEDOS': 4096,
'SIGMA': 0.01}},
'structure': 'SiC_0.cif',
'vasp_input_set': 'MinimalVaspInputSet'},
{'_fw_name': '{{pymatgen.io.vasp.interfaces.RunCustodianTask}}',
'handler_params': {},
'handlers': []}]}}
self.assertDictEqual(fw_dic, gdic, msg=None)
@unittest.skipUnless(1 if pkgutil.find_loader("fireworks") else 0, "Requires Fireworks")
class VaspWorkflowTest(unittest.TestCase):
def setUp(self):
self.mol_file = 'SiC_0.cif'
self.config_file = os.path.join(test_dir, 'vasp_interface_defaults.yaml')
self.input = VaspInputInterface(s=self.mol_file, config_file=self.config_file)
self.fw1 = VaspFirework(self.input, config_file=self.config_file)
self.fw2 = VaspFirework(self.input, config_file=self.config_file)
self.fw3 = VaspFirework(self.input, config_file=self.config_file)
self.links1 = {'-1': [-2], '-2': [-3], '-3': []}
self.links2 = {'-1': [-2, -3], '-2': [], '-3': []}
def tearDown(self):
pass
def test_make_seq_workflow_from_multi_fws(self):
wf1 = VaspWorkflow(self.fw1, self.fw2, self.fw3)
wf_dic = wf1.wf.as_dict()
ldic = wf_dic['links']
# Rebuild WF1 Dictionary to reflect FWs '-1', '-2', and '-3' instead
# of higher FW_ID numbers
min_fw_id = int(min(ldic.keys()))
modifier = -( min_fw_id + 1 )
link_dic = {str(min_fw_id + modifier): [ldic[str(min_fw_id)][0] + modifier],
str(min_fw_id + modifier - 1): [ldic[str(min_fw_id-1)][0] + modifier],
str(min_fw_id + modifier - 2): []}
self.assertDictEqual(link_dic, self.links1, msg=None)
def test_add_fw(self):
wf1 = VaspWorkflow(self.fw1)
wf1.add_fw(self.fw2)
wf1.add_fw(self.fw3)
wf_dic = wf1.wf.as_dict()
ldic = wf_dic['links']
# Rebuild WF1 Dictionary to reflect FWs '-1', '-2', and '-3' instead
# of higher FW_ID numbers
min_fw_id = int(min(ldic.keys()))
modifier = -( min_fw_id + 1 )
link_dic = {str(min_fw_id + modifier): [ldic[str(min_fw_id)][0] + modifier],
str(min_fw_id + modifier - 1): [ldic[str(min_fw_id-1)][0] + modifier],
str(min_fw_id + modifier - 2): []}
self.assertDictEqual(link_dic, self.links1, msg=None)
def test_multifw_wf_custom_deps(self):
wf1 = VaspWorkflow(self.fw1, self.fw2, self.fw3,
deps_dict={self.fw1: [self.fw2, self.fw3]})
wf_dic = wf1.wf.as_dict()
ldic = wf_dic['links']
# Rebuild WF1 Dictionary to reflect FWs '-1', '-2', and '-3' instead
# of higher FW_ID numbers
min_fw_id = int(min(ldic.keys()))
modifier = -( min_fw_id + 1 )
link_dic = {str(min_fw_id + modifier): [
ldic[str(min_fw_id)][0] + modifier, ldic[str(min_fw_id)][1] + modifier],
str(min_fw_id + modifier - 1): [],
str(min_fw_id + modifier - 2): []}
self.assertDictEqual(link_dic, self.links2, msg=None)
@unittest.skipUnless(1 if pkgutil.find_loader("fireworks") else 0, "Requires Fireworks")
class VaspTransferTaskTest(unittest.TestCase):
def setUp(self):
self.src_dir = os.path.join(test_dir, 'TRNFR_TEST_FILES')
self.dest_dir = os.path.join(os.environ['HOME'], 'TRNFR_TEST')
if not os.path.exists(self.dest_dir):
os.mkdir(self.dest_dir)
def tearDown(self):
os.chdir(MODULE_DIR)
if os.path.exists(self.dest_dir):
shutil.rmtree(self.dest_dir)
def test_file_transfer_fail1(self):
os.chdir(self.dest_dir)
fw_fail1 = VaspTransferTask(mode='copy', dir=self.src_dir,
files=['test_f1.txt', 'test_f2.txt', 'test_f3.txt'],
ignore_errors=False)
self.assertRaises(ValueError, fw_fail1.run_task, fw_spec={})
def test_file_transfer_fail2(self):
os.chdir(self.dest_dir)
fw_fail2 = VaspTransferTask(mode='copy',
files=['test_f1.txt'], ignore_errors=False)
self.assertRaises(RuntimeError, fw_fail2.run_task, fw_spec={})
def test_file_transfer_fail3(self):
os.chdir(self.dest_dir)
fw_fail3 = VaspTransferTask(mode='copy',
files=['test_f1.txt', 'test_f2.txt'],
ignore_errors=False)
self.assertRaises(TypeError, fw_fail3.run_task)
def test_file_transfer_succeed1(self):
fw_succ1 = VaspTransferTask(mode='copy', dir=self.src_dir,
files=['test_f1.txt', 'test_f2.txt', 'test_f3.txt'],
ignore_errors=True)
os.chdir(self.dest_dir)
fw_succ1.run_task(fw_spec={})
self.assertListEqual(os.listdir(self.dest_dir), os.listdir(self.src_dir))
def test_file_transfer_succeed2(self):
fw_succ2 = VaspTransferTask(mode='copy', dir=self.src_dir,
files=['test_f1.txt', 'test_f2.txt'],
ignore_errors=False)
os.chdir(self.dest_dir)
fw_succ2.run_task(fw_spec={})
self.assertListEqual(os.listdir(self.dest_dir), os.listdir(self.src_dir))
def test_file_transfer_from_previous(self):
fw1 = VaspTransferTask(mode='copy',
files=['test_f1.txt', 'test_f2.txt'],
ignore_errors=False)
os.chdir(self.dest_dir)
fw1.run_task(fw_spec= {'PREV_DIR': self.src_dir})
self.assertListEqual(os.listdir(self.dest_dir), os.listdir(self.src_dir))
if __name__ == '__main__':
unittest.main()
<file_sep>Change log
==========
v3.3.4
------
* Procar now supports parsing of phase factors.
* Miscellaneous bug fixes.
<file_sep>#!/usr/bin/env python
import os
import sys
import string
import json
from pymatgen import Structure
from fireworks import Firework, Workflow, LaunchPad
from pymatgen.io.vasp.interfaces import VaspInputInterface, VaspFirework, VaspWorkflow
from fireworks.user_objects.firetasks.script_task import ScriptTask
# get structure for use in VASP input
material='Si_example.cif'
s=Structure.from_file(material)
pf=s.formula
material_file_name="{}_tddft.yaml".format(pf)
mname="{}_tddft".format(pf)
print "creating VASP jobs specifications in ",material_file_name
# and make it dependent on the number of cores
ncores=512
kpar_dft=32
kpar_chi=32
ncore_per_kpoint_dft=ncores/kpar_dft
ncore_per_kpoint_chi=ncores/kpar_chi
remainder=ncore_per_kpoint_dft%ncore_per_kpoint_chi
fw1_task1=ScriptTask.from_str("pwd")
fw1=VaspFirework(fw1_task1,name=mname)
# standard DFT first
vasp1=VaspInputInterface(s)
nelect=vasp1.get_nelect()
nbands=nelect*3/2
remainder=nbands%ncore_per_kpoint_dft
add=ncore_per_kpoint_dft-remainder
nbands=int(nbands+add)
vasp1.ALGO='Normal'
vasp1.KPAR=kpar_dft
vasp1.NBANDS=nbands
vasp1.NEDOS=4096
vasp1.kpts_style='Gamma'
vasp1.kpts=[6,6,6]
vasp1.kpts_shift=[0,0,0]
fw1.add_task(vasp1)
fw1.add_handler('FrozenJobErrorHandler')
fw1_task2=ScriptTask.from_str("pwd; mkdir OrbDir")
fw1_task3=ScriptTask.from_str("mv CHG* CONTCAR DOSCAR EIGENVAL I* K* OSZICAR OUTCAR P* W* X* vasp* OrbDir")
fw1_task4=ScriptTask.from_str("cp OrbDir/WAVECAR . ; cp OrbDir/CONTCAR .")
fw1.add_task(fw1_task2)
fw1.add_task(fw1_task3)
fw1.add_task(fw1_task4)
# create VASP IPA LOPTICS input job yaml file
vasp2=VaspInputInterface('CONTCAR')
vasp2.ISTART=1
vasp2.ALGO='Normal'
vasp2.LHFCALC='.TRUE.'
vasp2.LOPTICS='True'
vasp2.CSHIFT=0.1
vasp2.ISTART=1
vasp2.HFSCREEN=0.2
vasp2.NEDOS=4096
vasp2.KPAR=kpar_dft
vasp2.NBANDS=nbands
vasp2.kpts=[6,6,6]
vasp2.kpts_shift=[0,0,0]
vasp2.kpts_style='Gamma'
vasp2.TIME="0.4"
vasp2.PRECFOCK="Fast"
vasp2.NKRED=3
fw1.add_task(vasp2)
# Create VASP LFE Optics input. This is for a new FireWorker
vasp3=VaspInputInterface('CONTCAR')
vasp3.ALGO='Chi'
vasp3.LHFCALC='.TRUE.'
vasp3.HFSCREEN=0.2
vasp3.LSPECTRAL='False'
vasp3.NEDOS=4096
vasp3.NOMEGA=1024
vasp3.NBANDS=nbands
vasp3.LOPTICS='False'
vasp3.LRPA='.FALSE.'
vasp3.LFXC='True'
vasp3.NKREDLFX=3
vasp3.NKREDLFY=3
vasp3.NKREDLFZ=3
vasp3.KPAR=kpar_chi
vasp3.kpts_style='Gamma'
vasp3.kpts=[6,6,6]
vasp3.kpts_shift=[0,0,0]
fw2=VaspFirework(vasp3, name=mname)
fw2.copy_files_from_previous('WAVECAR', 'WAVEDER', mode='copy', ignore_errors=True)
# Make Sequential Workflow
#wf1 = VASPWorkflow(fw1,fw2,fw3, name=material_file_name.split('.')[0])
wf=VaspWorkflow(fw1,fw2,name=material_file_name.split('.')[0])
wf.to_file(material_file_name)
# save specification to yaml file for later inspection
# or manual add to lauchpad with lpad script
#wf.add_wf_to_launchpad()
| 8e1012375203b3107175418661873595f2fd26f6 | [
"Python",
"reStructuredText"
] | 5 | Python | dcossey014/pymatgen | bed24cf3c36e5a7639cad4f6edd435adf72c78ca | 696c250b60ddb3b46e6b2263f38cde805ea3731c | |
refs/heads/master | <file_sep># seq2seq-haruka-amami-01
転移学習を用いた天海春香AI
## About
詳しくは[天海春香会話bot開発チャレンジ3 - かわみのメモ帳](http://kawami.hatenablog.jp/entry/2018/06/17/192429)を参照。
[赤間 怜奈, 稲田 和明, 小林 颯介, 佐藤 祥多, 乾 健太郎: 転移学習を用いた対話応答のスタイル制御, 言語処理学会第23回年次大会 発表論文集, pp.338-341, 2017](http://www.anlp.jp/proceedings/annual_meeting/2017/pdf_dir/B3-3.pdf)
で提案されている手法をそのままChainerで実装。
発話生成には一般的なEncoder-Decoderモデルを用い、事前学習とスタイル付与学習の際に一部語彙を入れ替え。
なお、この実装自体に特に天海春香要素はありません。
## How To Use
MeCab本体およびPythonバインディングをインストールし、config.ymlに値を設定したのち、
> python3 transfer.py
で動くと思われます。ただしお手数ですが、ソースコード中のTRAINの値をTrueにしないと学習モードになりません。GPUの設定も然り。
### config.yml
だいたい見ていただければどこに何の値を設定すれば良いのかお分かりかと思います。
ちなみに"max_vocab_size"は事前学習およびスタイル付与データを用いた学習で用いる最大語彙数、
"replaced_vocab_size"はスタイル付与データを用いた学習の際に入れ替える語彙数です。
<file_sep>import chainer
from chainer import links as L
from chainer import functions as F
from chainer import optimizers
from collections import Counter
import numpy as np
from chainer import cuda
import random
import math
from datetime import datetime
import yaml
from mecab_tokenizer import Tokenizer
import util
from logging import getLogger, StreamHandler, INFO, DEBUG
logger = getLogger(__name__)
handler = StreamHandler()
handler.setLevel(INFO)
logger.setLevel(INFO)
logger.addHandler(handler)
class EncoderDecoder(chainer.Chain):
def __init__(self, encoder_layers, decoder_layers, input_vocab_size, output_vocab_size, embed_size,
hidden_size, dropout, ARR):
super(EncoderDecoder, self).__init__()
with self.init_scope():
self.embed_input = L.EmbedID(in_size=input_vocab_size, out_size=embed_size)
self.embed_output = L.EmbedID(in_size=output_vocab_size, out_size=embed_size)
self.encoder = L.NStepLSTM(n_layers=encoder_layers, in_size=embed_size, out_size=hidden_size,
dropout=dropout)
self.decoder = L.NStepLSTM(n_layers=decoder_layers, in_size=embed_size, out_size=hidden_size,
dropout=dropout)
self.output = L.Linear(hidden_size, output_vocab_size)
self.ARR = ARR
def __call__(self, input, output):
input = [x[::-1] for x in input]
batch = len(input)
eos = self.ARR.array([EOS_TAG[1]], dtype=self.ARR.int32)
y_in = [F.concat([eos, y], axis=0) for y in output]
y_out = [F.concat([y, eos], axis=0) for y in output]
input_embed = [self.embed_input(i) for i in input]
output_embed = [self.embed_output(y) for y in y_in]
e_hx, e_cx, _ = self.encoder(cx=None, xs=input_embed, hx=None)
_, _, os = self.decoder(cx=e_cx, xs=output_embed, hx=e_hx)
op = self.output(F.concat(os, axis=0))
loss = F.sum(F.softmax_cross_entropy(op, F.concat(y_out, axis=0), reduce='no'))
return loss / batch
def test(self, input, max_length):
input = input[::-1]
input_embed = [self.embed_input(input)]
e_hx, e_cx, _ = self.encoder(cx=None, xs=input_embed, hx=None)
ys = self.ARR.array([EOS_TAG[1]], dtype=self.ARR.int32)
result = []
for i in range(max_length):
output_embed = [self.embed_output(ys)]
e_hx, e_cx, ys = self.decoder(cx=e_cx, xs=output_embed, hx=e_hx)
cys = F.concat(ys, axis=0)
os = self.output(cys)
ys = self.ARR.argmax(os.data, axis=1).astype(self.ARR.int32)
if ys == EOS_TAG[1]:
break
result.append(ys)
# logger.info(result)
if len(result) > 0:
result = cuda.to_cpu(self.ARR.concatenate([self.ARR.expand_dims(x, 0) for x in result]).T)
# logger.info(result)
return result
def get_pre_train_data(PRE_TRAIN_FILE, max_vocab_size):
"""
事前学習用データを取得
:param PRE_TRAIN_FILE:
:param max_vocab_size:
:return:
"""
with open(file=PRE_TRAIN_FILE[0], encoding="utf-8") as question_text_file, open(file=PRE_TRAIN_FILE[1],
encoding="utf-8") as answer_text_file:
answer_lines = answer_text_file.readlines()
question_lines = question_text_file.readlines()
vocab = []
for line1, line2 in zip(answer_lines, question_lines):
vocab.extend(line1.replace("\t", " ").split())
vocab.extend(line2.replace("\t", " ").split())
logger.debug(vocab)
vocab_counter = Counter(vocab)
vocab = [v[0] for v in vocab_counter.most_common(max_vocab_size)] # 語彙制限
word_to_id = {v: i + 2 for i, v in enumerate(vocab)}
word_to_id[UNKNOWN_TAG[0]] = UNKNOWN_TAG[1]
word_to_id[EOS_TAG[0]] = EOS_TAG[1]
id_to_word = {i: v for v, i in word_to_id.items()}
train_data = []
for question_line, answer_line in zip(question_lines, answer_lines):
if len(question_line) < 1 or len(answer_line) < 1:
continue
input_words = [word_to_id[word] if word in word_to_id.keys() else word_to_id[UNKNOWN_TAG[0]] for word in
question_line.split()]
output_words = [word_to_id[word] if word in word_to_id.keys() else word_to_id[UNKNOWN_TAG[0]] for word in
answer_line.split()]
if len(input_words) > 0 and len(output_words):
train_data.append((input_words, output_words))
return train_data, word_to_id, id_to_word
def get_style_train_data(STYLE_TRAIN_FILE, PRE_TRAIN_FILE, pre_word_to_id, pre_id_to_word):
"""
スタイル付与データを取得
:param STYLE_TRAIN_FILE:
:param PRE_TRAIN_FILE:
:param pre_word_to_id:
:param pre_id_to_word:
:return:
"""
style_vocab = []
pre_vocab = []
with open(STYLE_TRAIN_FILE, encoding="utf-8") as style_file, open(PRE_TRAIN_FILE[0],
encoding="utf-8") as pre_file1, open(
PRE_TRAIN_FILE[1], encoding="utf-8") as pre_file2:
style_lines = style_file.readlines()
pre_lines1 = pre_file1.readlines()
pre_lines2 = pre_file2.readlines()
for style_line, pre_line1, pre_line2 in zip(style_lines, pre_lines1, pre_lines2):
style_vocab.extend(style_line.replace("\t", " ").split())
pre_vocab.extend(pre_line1.split())
pre_vocab.extend(pre_line2.split())
pre_lines1 = pre_lines2 = None
# 単語の入れ替え
style_high_freq_words = restrict_dict(pre_vocab, style_vocab)
for i, style_high_freq_word in enumerate(style_high_freq_words):
pre_id_to_word[i + 2 + max_vocab_size - top_vocab_size] = style_high_freq_word
pre_word_to_id[style_high_freq_word] = i + 2 + max_vocab_size - top_vocab_size
train_data = []
for line in style_lines:
spl = line.split("\t")
if len(spl) < 2:
continue
input = spl[0]
output = spl[1]
input_words = [pre_word_to_id[word] if word in pre_word_to_id.keys() else pre_word_to_id[UNKNOWN_TAG[0]] for
word in input.split()]
output_words = [pre_word_to_id[word] if word in pre_word_to_id.keys() else pre_word_to_id[UNKNOWN_TAG[0]] for
word in output.split()]
if len(input_words) > 0 and len(output_words):
train_data.append((input_words, output_words))
return train_data, pre_word_to_id, pre_id_to_word
def restrict_dict(pre_vocab, style_vocab):
# 転移学習する側の辞書のみに含まれるN単語を取得
style_counter = Counter(style_vocab).items()
pre_counter = Counter(pre_vocab).most_common(max_vocab_size)
pre_rpl_vocab = [w for w, f in pre_counter[:-top_vocab_size]]
style_high_freq_words = set()
for w, f in style_counter:
if w not in pre_rpl_vocab:
style_high_freq_words.add(w)
if len(style_high_freq_words) == top_vocab_size:
break
return style_high_freq_words
def train(model, train_data, word_to_id, id_to_word, model_path, mode):
"""
学習
:param model:
:param train_data: 学習データ
:param word_to_id: 単語 -> 単語ID への変換用辞書
:param id_to_word: 単語ID -> 単語 への変換用辞書
:param model_path: モデルの保存先のパス
:param mode: 学習モード。ただ表示するだけ。
:return:
"""
logger.info(word_to_id)
vocab_size = len(id_to_word)
logger.info("========== MODE: %s ==========" % mode)
logger.info("========== vocab_size: %s ==========" % vocab_size)
logger.info("========== train_data_size:%s ==========" % len(train_data))
logger.debug(id_to_word)
util.save(word_to_id, model_path + "_w2i.pkl")
util.save(id_to_word, model_path + "_i2w.pkl")
optimizer = optimizers.Adam()
optimizer.setup(model)
optimizer.add_hook(chainer.optimizer.GradientClipping(10.0))
for epoch in range(epoch_num):
if GPU:
gpu_device = 0
cuda.get_device(gpu_device).use()
model.to_gpu(gpu_device)
total_loss = 0
logger.info("=============== EPOCH {} {} ===============".format(epoch + 1, datetime.now()))
length = math.ceil(len(train_data) / batch_size)
logger.debug(train_data)
for i in range(length):
logger.debug("===== {} / {} =====".format(i, length))
mini_batch = train_data[i * batch_size: (i + 1) * batch_size]
t = [xp.asarray(data[1], dtype=xp.int32) for data in mini_batch]
x = [xp.asarray(data[0], dtype=xp.int32) for data in mini_batch]
logger.debug("x:{} , y:{}".format(x, t))
loss = model(x, t)
total_loss += loss.data
model.cleargrads()
loss.backward()
loss.unchain_backward()
optimizer.update()
logger.debug("=============== loss: %s ===============" % loss)
logger.info("=============== total_loss: %s ===============" % total_loss)
test_input = xp.array(train_data[random.randint(0, len(train_data) - 1)][0], dtype=xp.int32)
test_result = model.test(test_input, SEQUENCE_MAX_LENGTH)
logger.info("=============== TEST ===============")
logger.info("input: %s" % str([id_to_word.get(int(id_), UNKNOWN_TAG[0]) for id_ in test_input]))
logger.debug(test_result)
logger.info("output: %s" % str([id_to_word.get(int(id_), UNKNOWN_TAG[0]) for id_ in test_result[0]]))
model.to_cpu()
chainer.serializers.save_npz(model_path, model)
def pre_train(PRE_TRAIN_FILE, PRE_MODEL_FILE):
"""
事前学習
:return:
"""
pre_train_data, pre_word_to_id, pre_id_to_word = get_pre_train_data(PRE_TRAIN_FILE, max_vocab_size)
vocab_size = len(pre_word_to_id)
pre_train_model = EncoderDecoder(encoder_layers=lstm_layers, decoder_layers=lstm_layers,
input_vocab_size=vocab_size, output_vocab_size=vocab_size, embed_size=embed_size,
hidden_size=hidden_size, dropout=dropout, ARR=xp)
train(model=pre_train_model, train_data=pre_train_data, word_to_id=pre_word_to_id, id_to_word=pre_id_to_word,
model_path=PRE_MODEL_FILE, mode="PRE_TRAIN")
return pre_word_to_id, pre_id_to_word
def transfer_train(PRE_TRAIN_FILE, STYLE_TRAIN_FILE, STYLE_MODEL_FILE, PRE_MODEL_FILE, pre_word_to_id, pre_id_to_word):
"""
転移学習
:return:
"""
style_train_data, style_word_to_id, style_id_to_word = get_style_train_data(STYLE_TRAIN_FILE, PRE_TRAIN_FILE,
pre_word_to_id, pre_id_to_word)
vocab_size = len(style_id_to_word)
pre_train_model = EncoderDecoder(encoder_layers=lstm_layers, decoder_layers=lstm_layers,
input_vocab_size=vocab_size, output_vocab_size=vocab_size, embed_size=embed_size,
hidden_size=hidden_size, dropout=dropout, ARR=xp)
chainer.serializers.load_npz(PRE_MODEL_FILE, pre_train_model)
train(model=pre_train_model, train_data=style_train_data, word_to_id=style_word_to_id, id_to_word=style_id_to_word,
model_path=STYLE_MODEL_FILE, mode="STYLE_TRAIN")
def tokenize():
"""
入力に使うデータをすべて形態素解析
:return:
"""
logger.info("========= START TO TOKENIZE ==========")
tokenizer = Tokenizer("-Ochasen -d " + neologd_dic_path)
# 事前学習用データの形態素解析
pre_train_tokenized = []
with open(PRE_TRAIN_FILE[0], encoding="utf-8") as f1, open(PRE_TRAIN_FILE[1], encoding="utf-8") as f2:
for line1, line2 in zip(f1.readlines(), f2.readlines()):
if len(line1) < 1 or len(line2) < 1:
continue
try:
pre_train_tokenized.append((" ".join([token.surface for token in tokenizer.tokenize(line1)]),
" ".join([token.surface for token in tokenizer.tokenize(line2)])))
except: # 形態素解析でエラー発生の場合
pass
with open(tmp_dir + "pretrain_input.txt", "wt", encoding="utf-8") as f1, open(tmp_dir + "pretrain_output.txt", "wt",
encoding="utf-8") as f2:
for line1, line2 in pre_train_tokenized:
f1.write(line1 + "\r\n")
f2.write(line2 + "\r\n")
pre_train_tokenized = None
# 転移学習用データの形態素解析
style_train_tokenized = []
with open(STYLE_TRAIN_FILE, encoding="utf-8") as f:
for line in f.readlines():
spl = line.split("\t")
if len(spl) < 2 or len(spl[0]) < 1 or len(spl[1]) < 1:
continue
try:
style_train_tokenized.append(
(" ".join([token.surface for token in tokenizer.tokenize(spl[0])])) + "\t" +
" ".join([token.surface for token in tokenizer.tokenize(spl[1])]) + "\r\n")
except:
pass
with open(tmp_dir + "style_data.txt", "wt", encoding="utf-8") as f:
f.writelines(style_train_tokenized)
logger.info("========= FINISH TO TOKENIZE ==========")
def start():
# まず形態素解析
# tokenize()
# 事前学習
pre_word_to_id, pre_id_to_word = pre_train((tmp_dir + "pretrain_input.txt", tmp_dir + "pretrain_output.txt"),
PRE_MODEL_FILE)
# 転移学習
transfer_train((tmp_dir + "pretrain_input.txt", tmp_dir + "pretrain_output.txt"), tmp_dir + "style_data.txt",
STYLE_MODEL_FILE, PRE_MODEL_FILE, pre_word_to_id, pre_id_to_word)
class Predict:
"""
学習済みモデルを用いた予測に使うクラス
"""
def __init__(self, model_path):
self.word_to_id = util.load(model_path + "_w2i.pkl")
self.id_to_word = util.load(model_path + "_i2w.pkl")
vocab_size = len(self.id_to_word)
model = EncoderDecoder(encoder_layers=lstm_layers, decoder_layers=lstm_layers,
input_vocab_size=vocab_size, output_vocab_size=vocab_size,
embed_size=embed_size,
hidden_size=hidden_size, dropout=dropout, ARR=np)
chainer.serializers.load_npz(model_path, model)
self.model = model
def predict(self, input_words, maxlength):
input = np.asarray([self.word_to_id.get(w, UNKNOWN_TAG[1]) for w in input_words], dtype=np.int32)
y = self.model.test(input, maxlength)
return [self.id_to_word.get(id, UNKNOWN_TAG[0]) for id in y[0]]
TRAIN = False
GPU = False
xp = cuda.cupy if GPU else np
SEQUENCE_MAX_LENGTH = 50
config = yaml.load(open("config.yml", encoding="utf-8"))
PRE_TRAIN_FILE = (config["pre_train_file"]["q"], config["pre_train_file"]["a"])
STYLE_TRAIN_FILE = config["style_train_file"]
PRE_MODEL_FILE = config["encdec"]["pre_model"]
STYLE_MODEL_FILE = config["encdec"]["style_model"]
epoch_num = int(config["encdec"]["epoch"])
batch_size = int(config["encdec"]["batch"])
embed_size = int(config["encdec"]["embed"])
hidden_size = int(config["encdec"]["hidden"])
dropout = float(config["encdec"]["dropout"])
lstm_layers = int(config["encdec"]["lstm_layers"])
max_vocab_size = int(config["encdec"]["max_vocab_size"])
top_vocab_size = int(config["encdec"]["replaced_vocab_size"])
neologd_dic_path = config["neologd_dic_path"]
tmp_dir = config["tmp_dir"]
UNKNOWN_TAG = ("<UNK>", 0)
EOS_TAG = ("<EOS>", 1)
if __name__ == '__main__':
if TRAIN:
start()
else:
tokenizer = Tokenizer("-Ochasen -d " + neologd_dic_path)
predictor = Predict(STYLE_MODEL_FILE)
while True:
input_ = input("INPUT>>>")
output = "".join(
predictor.predict([token.surface for token in tokenizer.tokenize(input_)], SEQUENCE_MAX_LENGTH))
print("OUTPUT>>>%s" % output)
<file_sep>import MeCab
class Token:
def __init__(self, node):
self.surface = node.surface
self.__set_pos(node.feature)
def __set_pos(self, feature):
pos_list = feature.split(",")
self.base = pos_list[6]
self.reading = "" if len(pos_list) < 8 else pos_list[7]
detail_pos_list = []
for pos in pos_list:
if pos == "*":
break
detail_pos_list.append(pos)
self.pos = ",".join(detail_pos_list)
def __eq__(self, other):
return self.surface == other.surface and self.pos == other.pos
def __hash__(self):
return hash(self.surface + self.pos)
def __str__(self):
return self.surface
class Tokenizer:
def __init__(self, arg="mecabrc"):
self.t = MeCab.Tagger(arg)
def __parse(self, node):
token_list = []
while node:
token = Token(node)
if not token.pos.startswith("BOS/EOS"):
token_list.append(token)
node = node.next
return token_list
def tokenize(self, sentence):
self.t.parse("") # これをしないと正しい結果が返ってこないバグが存在
node = self.t.parseToNode(sentence)
return self.__parse(node)
if __name__ == '__main__':
neologd_dic_path = "/hogehoge/mecab-ipadic-neologd" # お好きな辞書のパス
test = "夜の水面に飛び交う蛍が流れ星みたいで綺麗なのん。のんのんびより。"
tokenizer = Tokenizer("-Ochasen -d " + neologd_dic_path)
token_list = tokenizer.tokenize(test)
for token in token_list:
print(token.surface + " : " + token.pos + " : " + token.base + " : " + token.reading)
<file_sep>import pickle
def save(obj, filename):
with open(filename, "wb") as f:
pickle.dump(obj, f)
def load(filename):
with open(filename, "rb") as f:
return pickle.load(f)
| f57f086b76b1430bf4c938680523933cc10d62d7 | [
"Markdown",
"Python"
] | 4 | Markdown | kawamix/seq2seq-haruka-amami-01 | 32aebf40fcbc641d370dc1370eb1effd7f3846b8 | 956c0727acf2329d6f0367dcdb4652866b057da4 | |
refs/heads/master | <repo_name>shiv7477/social<file_sep>/README.md
# social
social training repository
<file_sep>/shiv.java
class One
{
public static void main(string [] args)
{
System.out.println("My name is: Shivanand");
System.out.println("My roll no is: A31");
}
}
<file_sep>/k.java
class Simple{
public static void main(String args[]){
System.out.println("I am Akshit");
System.out.println("11711458");
}
}
| 89795e1e734d1beb60bc0e1c12bd84a727bab3b7 | [
"Markdown",
"Java"
] | 3 | Markdown | shiv7477/social | 0ffd6a0a1c294a6e5caa57d867b19b210f1faa91 | 5247f8fc05e6e0a50d5fd4150364463c5d02728e | |
refs/heads/master | <file_sep>---
id: classic-ha-queues
title: Classic Ha Queues (aka Mirrored Queues)
sidebar_label: Classic Ha Queues
---
TODO<file_sep>---
id: queue-host
title: Queue Host Awareness
---
TODO<file_sep>---
id: classic-vs-replicated
title: Classic vs Replicated Queues
---
TODO<file_sep>---
id: metrics
title: Metrics
---
Metric collection and monitoring are as important for publishers as they are for any other application or component in an application. Several metrics collected by RabbitMQ are of particular interest when it comes to publishers:
- Outgoing message rate
- Publisher confirmation rate
- Connection churn rate
- Channel churn rate
- Unroutable dropped message rate
- Unroutable returned message rate
The publishing and confirmation rates are mostly self-explanatory. The churn rates are so important because they help detect applications that do not use connections or channels optimally and thus offer sub-optimal publishing rates and waste resources.
Unroutable message rates can help detect applications that publish messages that cannot be routed to any queue. For example, this may suggest a misconfiguration.
Client libraries may also collect metrics. RabbitMQ Java client is one example. These metrics can provide insight into application-specific architecture (e.g. what publishing component publishes unrooutable messages) that RabbitMQ nodes cannot infer.<file_sep>---
id: channels
title: Channels
---
TODO<file_sep>---
id: federated-exchanges
title: Federated Exchanges
---
TODO<file_sep>---
id: queues
title: Queues
---
A queue stores messages in FIFO order until they can be delivered to a consumer. Messages are deleted either once the consumer acknowledges them or auto-acknowledgements are used.
Likewise, publishers can request confirmation that their messages have been persisted to one or more queues (called Publisher Confirms). These publisher confirms and consumer acknowledgements form the basis of reliable messaging in RabbitMQ.
If there is currently no consumer then the message remains in the queue until consumption (see note).
> NOTE: Messages and queues can be configured to be durable or non-durable and even ephemeral with auto-delete features. See the Reliability Guide for an introduction to message durability.
## Queue Types
There are three types of queue available.
### Classic queue
This is an unreplicated queue that exists only on a single broker. Use this queue when you only have a single broker or high availability of the queue is not required. Classic queues offer the highest throughput and lowest latency of the queue types.
### Classic HA queue
This is a replicated queue that can exist across a cluster of brokers. It is designed to provide safety against data loss and provide high availability. See the High Availabilty page to learn more.
### Quorum queue
This is a replicated queue designed to replace Classic HA queues, based on the Raft protocol. It is the recommend queue type for when you need high availability of a queue and strong guarantees against data loss.
## Queue Configurations
### Limits
We can configure queues to not grow beyond a certain size or length via the use of policies. When a queue becomes "full" it will start either rejecting, discarding or moving messages into an overflow queue, according to what you configure it to do.
### Time-to-live (TTL)
Classic and Classic HA queues allow for messages to be discarded once they exceed a given time-limit
### Lazy Mode
Classic and Classic HA queues by default will try and keep messages in memory. This can cause memory issues when queues grow very large. When a queue is configured to be lazy it will remove messages from memory once successfully written to disk and lazily load them back into memory on demand. This lowers the memory footprint dramatically.<file_sep>---
id: robust-systems
title: Characteristics of Robust Systems
---
TODO<file_sep>---
id: safety-in-flight
title: Safety In-Flight
---
TODO<file_sep>---
id: client-libraries
title: Client Libraries
---
TODO<file_sep>---
id: start-rabbitmq
title: Start RabbitMQ
---
This series of Getting Started steps considers that you already
[installed RabbitMQ](../install/).
The very first thing will be to start RabbitMQ. Once this is done, we
will:
1. use its CLI (command line interface) to verify it started
successfully and
2. ensure we can communicate with it.
## Starting the broker
The way to do it really depends on the method you used to install it.
This article will give a few common examples. For more informations,
please read the documentation of your package manager or deployment
tool. It is even possible that RabbitMQ was started for you already.
### If you use the generic-unix package
Starting RabbitMQ is as simple as running the rabbitmq-server(8) script,
located in `sbin`, in the directory created by decompressing the
generic-unix archive:
```sh
/path/to/rabbitmq_server-$version/sbin/rabbitmq-server
```
This command will display the following banner in the terminal:
```text
## ## RabbitMQ 3.8.2
## ##
########## Copyright (c) 2007-2019 Pivotal Software, Inc.
###### ##
########## Licensed under the MPL 1.1. Website: https://rabbitmq.com
Doc guides: https://rabbitmq.com/documentation.html
Support: https://rabbitmq.com/contact.html
Tutorials: https://rabbitmq.com/getstarted.html
Monitoring: https://rabbitmq.com/monitoring.html
Logs: /tmp/rabbitmq_server-3.8.2/var/log/rabbitmq/[email protected]
/tmp/rabbitmq_server-3.8.2/var/log/rabbitmq/rabbit@cassini_upgrade.log
Config file(s): (none)
Starting broker... completed with 0 plugins.
```
### On most Unix-like systems with a package manager
The package probably installed RabbitMQ as a service. Therefore, use the
tool on your system to start the corresponding service:
* On Linux distributions based on systemd:
```sh
systemctl start rabbitmq-server
```
* On Linux distributions based on SysV init scripts:
```sh
service rabbitmq-server start
```
* On FreeBSD:
```sh
service rabbitmq start
```
### On Microsoft Windows
RabbitMQ is installed as a service which is started automatically. There
is nothing to do in particular.
## Checking the broker is running
### Using the command line interface
Now that RabbitMQ is supposedly running, you can use the CLI to query
its status:
```sh
rabbitmqctl status
```
This status command is quite verbose. Here is an example based on the
generic-unix-based RabbitMQ started earlier:
```text
Status of node rabbit@cassini ...
Runtime
OS PID: 19392
OS: FreeBSD
Uptime (seconds): 16
RabbitMQ version: 3.8.2
Node name: rabbit@cassini
Erlang configuration: Erlang/OTP 21 [erts-10.3.5.9] [source] [64-bit] [smp:8:8] [ds:8:8:10] [async-threads:128] [hipe] [dtrace]
Erlang processes: 271 used, 1048576 limit
Scheduler run queue: 1
Cluster heartbeat timeout (net_ticktime): 60
Plugins
Enabled plugin file: /tmp/rabbitmq_server-3.8.2/etc/rabbitmq/enabled_plugins
Enabled plugins:
Data directory
Node data directory: /tmp/rabbitmq_server-3.8.2/var/lib/rabbitmq/mnesia/rabbit@cassini
Config files
Log file(s)
* /tmp/rabbitmq_server-3.8.2/var/log/rabbitmq/[email protected]
* /tmp/rabbitmq_server-3.8.2/var/log/rabbitmq/rabbit@cassini_upgrade.log
(...)
Listeners
Interface: [::], port: 25672, protocol: clustering, purpose: inter-node and CLI tool communication
Interface: [::], port: 5672, protocol: amqp, purpose: AMQP 0-9-1 and AMQP 1.0
Interface: 0.0.0.0, port: 5672, protocol: amqp, purpose: AMQP 0-9-1 and AMQP 1.0
```
There are several CLI tools provided with RabbitMQ to interact with it:
* rabbitmqctl(8)
* rabbitmq-diagnostics(8)
* rabbitmq-plugins(8)
* rabbitmq-queues(8)
* rabbitmq-upgrade(8)
We will use some of them later in this guide.
### Using log files
The RabbitMQ log files location depends again on how you installed it.
The exact location is reported by `rabbitmqctl status` as seen above.
Common locations are:
* generic-unix package: `/path/to/rabbitmq_server-$version/var/log/rabbitmq`
* Linux/FreeBSD packages: `/var/log/rabbitmq`
* Microsoft Windows: `%APPDATA%\RabbitMQ\log`
Here is an example of the log file based on the generic-unix-based
RabbitMQ started earlier:
```sh
cat /tmp/rabbitmq_server-3.8.2/var/log/rabbitmq/[email protected]
```
```text
2020-02-13 20:17:38.236 [info] <0.259.0>
Starting RabbitMQ 3.8.2 on Erlang 172.16.17.32
Copyright (c) 2007-2019 Pivotal Software, Inc.
Licensed under the MPL 1.1. Website: https://rabbitmq.com
2020-02-13 20:17:38.237 [info] <0.259.0>
node : rabbit@cassini
home dir : /home/dumbbell
config file(s) : (none)
cookie hash : JD0UL5ltCvNhzE8sE1hEnw==
log(s) : /tmp/rabbitmq_server-3.8.2/var/log/rabbitmq/[email protected]
: /tmp/rabbitmq_server-3.8.2/var/log/rabbitmq/rabbit@cassini_upgrade.log
database dir : /tmp/rabbitmq_server-3.8.2/var/lib/rabbitmq/mnesia/rabbit@cassini
(...)
2020-02-13 20:17:38.438 [info] <0.595.0> started TCP listener on [::]:5672
2020-02-13 20:17:38.438 [info] <0.610.0> started TCP listener on 0.0.0.0:5672
2020-02-13 20:17:38.438 [info] <0.259.0> Running boot step cluster_name defined by app rabbit
2020-02-13 20:17:38.438 [info] <0.259.0> Running boot step direct_client defined by app rabbit
2020-02-13 20:17:38.438 [notice] <0.104.0> Changed loghwm of /tmp/rabbitmq_server-3.8.2/var/log/rabbitmq/[email protected] to 50
2020-02-13 20:17:38.519 [info] <0.8.0> Server startup complete; 0 plugins started.
```
<file_sep>---
id: delayed-messaging
title: Delayed Message Routing
---
TODO<file_sep>---
id: cluster-nodes
title: Cluster nodes
---
After tuning RabbitMQ to work well with your need, you probably want
to increase its reliability and fault tolerance by adding more nodes.
This tutorial will show you how to cluster three nodes and use a quorum
queue.
> **TODO**: Write this page.
<file_sep>---
id: microservice-frameworks
title: Microservice Frameworks
---
TODO<file_sep>---
id: rpc-routing
title: RPC / Reply To Queues
---
TODO<file_sep>---
id: sharding
title: Sharding
---
Explain Sharding Plugin, consistent hash exchange, random exchange
TODO<file_sep>---
id: index
title: Concepts
sidebar_label: Concepts Home
---
<div class="doc-tiles"></div>
* [](communication-patterns)
## [Communication Patterns](communication-patterns)
Pub/Sub, Point-to-point, Request-Reply...
* [](rabbitmq-way)
## [The RabbitMQ Way](rabbitmq-way)
Exchanges, queues, bindings...
* [](reliable-messaging)
## [Reliable Messaging](reliable-messaging)
Acknowledgements, durability...
* [](high-availability)
## [High Availability](high-availability)
Clusters, replicated queues...
* [](protocols)
## [Supported Protocols](protocols)
MQTT, STOMP, AMQP 1.0...
* [](streaming-across-clusters)
## [Cross-Cluster Streaming](streaming-across-clusters)
Multi-cluster architectures, edge computing and more...
<file_sep>---
id: connections
title: Connections
---
TODO<file_sep>---
title: We love Free Software!
author: <NAME>
---
The RabbitMQ project and team relies a **lot** on Free and Open Source
Software! From [Erlang](https://www.erlang.org/), the programming
language RabbitMQ is written in to [Docusaurus](https://docusaurus.io/),
the static website generator, to [Git](https://git-scm.com/), [GNU
Make](https://www.gnu.org/software/make/) and all the tools that make
our developers' life as seemless as possible.
A huge Thank you to all the amazing people behind those projects!

<file_sep>---
id: classic-queues
title: Classic Queues
---
TODO<file_sep># Testing Docusaurus for rabbitmq.com website
## How to run local webserver
```sh
cd website
yarn start
```
<file_sep>---
id: index
title: Welcome to RabbitMQ Docs
sidebar_label: Welcome to RabbitMQ Docs
---
<div class="doc-tiles"></div>
* [](intro)
## [Why use RabbitMQ?](intro)
Get an overview of the benefit of using RabbitMQ.
* [](../install/)
## [Install](../install/)
How install RabbitMQ<br/>
(or simply test it without installing).
* [](../getting-started/)
## [Getting started](../getting-started/)
Getting started with all RabbitMQ important features!
* [](../concepts/)
## [Concepts](../concepts/)
How message routing works in general.
* [](../enterprise/)
## [Enterprise Edition](../enterprise/)
The version of RabbitMQ with more advanced features.
* [](../developer-guide/)
## [Developer guide](../developer-guide/)
Documentation of RabbitMQ targetted at developers.
* [](../operations-guide/)
## [Operations guide](../operations-guide/)
Documentation of RabbitMQ targetted at admins.
<file_sep>---
id: work-orchestration
title: Work Orchestration Frameworks
---
TODO<file_sep>---
id: redhat
title: Install on Red Hat and derivatives
sidebar_label: Red Hat
---
> **TODO**: Write this page.
<file_sep>---
id: public-private-exchange
title: Public Message Exchange, Private Consumer Exchange
sidebar_label: Public-Private Exchange
---
TODO<file_sep>// vim:sw=2:et:
function add_main_menu_button() {
var breadcrumb_el = document.createElement('div');
breadcrumb_el.setAttribute("class", "navToggle");
breadcrumb_el.setAttribute("id", "navigationToggler");
breadcrumb_el.innerHTML =
"<div class=\"hamburger-menu\">" +
"<div class=\"line1\"></div>" +
"<div class=\"line2\"></div>" +
"<div class=\"line3\"></div></div>";
var sibling = document.querySelectorAll('.navigationWrapper')[0];
sibling.parentNode.insertBefore(breadcrumb_el, sibling);
function createToggler(togglerSelector, targetSelector, className) {
var toggler = document.querySelector(togglerSelector);
var target = document.querySelector(targetSelector);
if (!toggler) {
return;
}
toggler.onclick = function(event) {
event.preventDefault();
target.classList.toggle(className);
};
}
createToggler(
'#navigationToggler',
'.navigationWrapper',
'navigationSliderActive');
}
window.onload = add_main_menu_button;
<file_sep>---
id: erlang
title: Erlang/OTP
---
RabbitMQ relies on the Erlang/OTP runtime to run. A specific version of
RabbitMQ will be able to run on a range of Erlang/OTP versions. This
page documents that range for each version of RabbitMQ.
## Current requirements
As of **RabbitMQ 3.7.19+**, the requirements are:
<div class="erlang-requirements"></div>
* ### Minimum
21.3
* ### Maximum
22.x
## Installing Erlang/OTP
### From a package or installer
Please refer to the the install guide of RabbitMQ for your environment,
referenced in the left sidebar: it explains how to install Erlang/OTP.
### From source (Unix-like only)
The easiest way to compile and install Erlang/OTP from source as an
unprivileged user is to use [kerl].
Here is a quick overview of its usage:
1. Fetch the `kerl` CLI and make it executable:
```sh
wget https://raw.githubusercontent.com/kerl/kerl/master/kerl
chmod a+x kerl
```
2. Build a specific version of Erlang:
```sh
./kerl build 22.2 22.2
```
3. Install Erlang/OTP:
```sh
./kerl install 22.2 ~/kerl/22.2
```
4. Add `~/kerl/$erlang_version` to your `$PATH`.
For more details, please read [kerl documentation].
[kerl]: https://github.com/kerl/kerl/
[kerl documentation]: https://github.com/kerl/kerl/blob/master/README.md
## Compatibility matrix
| RabbitMQ version | Min. required Erlang/OTP | Max. supported Erlang/OTP |
|------------------|--------------------------|---------------------------|
| 3.8.2 | 21.3 | 22.x |
| 3.8.1 | 21.3 | 22.x |
| 3.8.0 | 21.3 | 22.x |
| 3.7.24 | 21.3 | 22.x |
| 3.7.23 | 21.3 | 22.x |
| 3.7.22 | 21.3 | 22.x |
| 3.7.21 | 21.3 | 22.x |
| 3.7.20 | 21.3 | 22.x |
| 3.7.19 | **21.3** | 22.x |
| 3.7.18 | 20.3 | 22.x |
| 3.7.17 | 20.3 | 22.x |
| 3.7.16 | 20.3 | 22.x |
| 3.7.15 | 20.3 | **22.x** |
| 3.7.14 | 20.3 | 21.3.x |
| 3.7.13 | 20.3 | 21.3.x |
| 3.7.12 | 20.3 | 21.3.x |
| 3.7.11 | **20.3** | **21.3.x** |
| 3.7.10 | 19.3 | 21.x |
| 3.7.9 | 19.3 | 21.x |
| 3.7.8 | 19.3 | 21.x |
| 3.7.7 | 19.3 | **21.x** |
| 3.7.6 | 19.3 | 20.3.x |
| 3.7.5 | 19.3 | 20.3.x |
| 3.7.4 | 19.3 | 20.3.x |
| 3.7.3 | 19.3 | 20.3.x |
| 3.7.2 | 19.3 | 20.3.x |
| 3.7.1 | 19.3 | 20.3.x |
| 3.7.0 | 19.3 | 20.3.x |
## Support Erlang/OTP versions policy
[Starting in January 2019][erlang-support-policy-ann], RabbitMQ supports
two most recent Erlang/OTP release series.
When a new Erlang/OTP release series is published, support for up to
three release series is provided for a few months:
* to allow RabbitMQ to stabilize on the new release, and
* for end users to upgrade their copy of Erlang if they are using the
minimum required version.
[erlang-support-policy-ann]: https://groups.google.com/d/msg/rabbitmq-users/G4UJ9zbIYHs/qCeyjkjyCQAJ
<file_sep>---
id: freebsd
title: Install on FreeBSD
sidebar_label: FreeBSD
---
> **TODO**: Write this page.
<file_sep>---
id: retry-messaging
title: Retry Message Routing
---
TODO<file_sep>---
title: This Month in RabbitMQ: January 2020
author: <NAME>
---
Introducing TGI RabbitMQ! Inspired by TGI Kubernetes, RabbitMQ engineer, <NAME> has begun a
series of tutorial videos. Tune in at the end of each month for the latest release. In January,
Gerhard covered [upgrading from 3.7 to 3.8](https://youtu.be/DynCqFtnSoY).
Star and watch the [repository](https://youtu.be/DynCqFtnSoY) for future episode updates.
Also, be sure to check out the [dashboards we’ve published to Grafana](https://grafana.com/orgs/rabbitmq). These are a great way to get started with the new [Prometheus and Grafana support in 3.8](https://www.rabbitmq.com/prometheus.html).
<!--truncate-->
## Project Updates
* First public beta version of [Pivotal RabbitMQ for Kubernetes](https://network.pivotal.io/products/p-rabbitmq-for-kubernetes).
* [Spring AMQP 2.2.3](https://github.com/spring-projects/spring-amqp/releases)
* [Hop 3.6.0](https://groups.google.com/d/msg/rabbitmq-users/MEwbDJ8biRc/s4xLPpvYAwAJ) is released with new features and dependency upgrades. The HTTP client for the classic blocking client is now pluggable, making it possible to use OkHttp instead of Apache HTTPComponents.
## Community Writings and Resources
* 1 Jan: <NAME> (@hsunryou) wrote about [installing RabbitMQ 3.8 on CentOS 8.0](https://blog.naver.com/hsunryou/221756168969)
* 1 Jan: (@Cakazies1) published about [messaging in Golang with RabbitMQ](https://medium.com/@cakazies/messaging-golang-with-rabbitmq-2ed1ccf8314)
* 4 Jan: <NAME> published a [beginners description](https://dev.to/deciduously/correct-a-beginner-about-buzzword-technologies-4bbe) of several “buzzword-y” technologies, including RabbitMQ, looking for feedback and corrections
* 8 Jan: <NAME> (@SunnyPaxos) published a really interesting article about [how DigitalOcean re-architected a system](https://blog.digitalocean.com/from-15-000-database-connections-to-under-100-digitaloceans-tale-of-tech-debt/) that had grown to 15,000 database connections because they were using MySQL as an event queue, to using RabbitMQ
* 8 Jan: <NAME> (@rmoff) wrote about [Streaming messages from RabbitMQ into Kafka](https://rmoff.net/2020/01/08/streaming-messages-from-rabbitmq-into-kafka-with-kafka-connect/) with Kafka Connect
* 9 Jan: <NAME> (@DormainDrewitz) published a list of the [top 10 RabbitMQ Influencers](https://content.pivotal.io/blog/top-rabbitmq-influencers-of-2019) of 2019
* 9 Jan: <NAME> (@szymonmentel) published a [5 minute video overview of RabbitMQ](https://youtu.be/ViJNPnZPJn4) along with a [post with notes and links](https://szkolarabbita.pl/rabbitmq-klaster-w-5-minut/) to try it yourself (in Polish)
* 9 Jan: <NAME> (@iandarigun) published the first part of an [introduction to RabbitMQ (in Portuguese)](https://medium.com/dev-cave/rabbit-mq-parte-i-c15e5f89d94)
* 10 Jan: <NAME> (@GSantomaggio) added Helm support for [his RabbitMQ Operator for Kubernetes](https://github.com/Gsantomaggio/rabbitmq-operator#install-the-rabbitmq-operator-with-helm3)
* 12 Jan: <NAME> (@pawel_duda) wrote about [why queues can be empty after a restart](https://devmeetsbiz.business.blog/2020/01/12/rabbitmq-queue-empty-after-a-restart-why-even-though-its-durable/) and what to do about it
* 13 Jan: <NAME> (@marcin_elem84) wrote about [confirming messages in RabbitMQ](https://czterytygodnie.pl/potwierdzanie-wiadomosci-rabbitmq/) (in Polish)
* 13 Jan: <NAME> (@DavideGuida82) wrote about using the [Outbox pattern with RabbitMQ](https://www.davideguida.com/improving-microservices-reliability-part-2-outbox-pattern/) to improve microservices reliability
* 15 Jan: <NAME> (@MikeMoelNielsen) published a [video on using a RabbitMQ PerfTest](https://youtu.be/MEdPLX-PCn8 ) (performance testing tool)
* 15 Jan: <NAME> (@iandarigun) published the second part of [an introduction to RabbitMQ in Portuguese](https://medium.com/dev-cave/rabbitmq-parte-ii-fa61a469ba2)
* 16 Jan: <NAME> (@TejadaRenzo) published about [using Docker Compose with RabbitMQ](https://renzotejada.com/blog/docker-compose-para-rabbitmq/) (in Spanish)
* 17 Jan: Linux Conference Australia 2020 published a talk by <NAME> (@rafaelma_) on what goes on behind the scenes of an ELK system, including the role of RabbitMQ ([video](https://youtu.be/4X0bmnb4tVI), [slides](https://e-mc2.net/behind-scenes-elk-system))
* 17 Jan: <NAME> (@lillajja) wrote up the [Annual RabbitMQ Report 2020](https://www.cloudamqp.com/blog/2020-01-17-annual-rabbitmq-report-2020-by-cloudamqp.html) from CloudAMQP
* 17 Jan: <NAME> (@SamProgramiz) published an [introduction to AMQP 0.9.1](https://medium.com/@samuelowino43/advanced-message-queueing-protocol-ampq-0-9-1-617209d2d6ec), which is one of the protocols supported in RabbitMQ
* 18 Jan: <NAME> wrote up [installing RabbitMQ on Windows](https://beetechnical.com/windows/rabbitmq-installation-on-windows/)
* 25 Jan: <NAME> published an introduction to [using RabbitMQ with Node.js](https://medium.com/@klogic/introduction-to-rabbitmq-with-nodejs-3f1ab928ed50)
* 27 Jan: <NAME> (@recursivecodes) published about [getting started with RabbitMQ in Oracle Cloud](https://blogs.oracle.com/developers/getting-started-with-rabbitmq-in-the-oracle-cloud)
* 27 Jan: <NAME> (@recursivecodes) also published a video about how to install and [run RabbitMQ in Oracle Cloud](https://youtu.be/9kVBZ5MQV6I)
* 27 Jan: <NAME> wrote an explainer about [RabbitMQ as a Service Bus in the context of ASP.NET Core](https://www.c-sharpcorner.com/article/rabbitmq-service-bus/)
* 28 Jan: <NAME> (@rseroter) wrote about trying out the new [replicated, durable quorum queues in RabbitMQ 3.8](https://seroter.wordpress.com/2020/01/28/lets-try-out-the-new-durable-replicated-quorum-queues-in-rabbitmq/)
* 28 Jan: <NAME> published about [using the Outbox pattern with RabbitMQ at Trendyol](https://medium.com/trendyol-tech/outbox-pattern-story-at-trendyol-fcb35fe056d7)
* 29 Jan: L<NAME> (@lillajja) wrote about [message priority in RabbitMQ](https://www.cloudamqp.com/blog/2020-01-29-message-priority-in-rabbitmq.html)
* 30 Jan: <NAME> (@T00MEKE) published about [setting up a RabbitMQ cluster in Kubernetes](https://nick.barrett.org.nz/setting-up-rabbitmq-ha-in-kubernetes-with-external-https-and-amqps-access-1ce1f3632dd2) with external HTTPS and AMQPS access
* 30 Jan: <NAME> (@lillajja) wrote about [RabbitMQ and Erlang upgrades](https://www.cloudamqp.com/blog/2020-01-30-rabbitmq-erlang-upgrades.html)
* 31 Jan: Georgy @georgysay wrote about [using RabbitMQ with .NET Core](https://habr.com/ru/post/486416/) (in Russian)
* 31 Jan: <NAME> (@gerhardlazu) published the first episode of TGI RabbitMQ on [how to upgrade from RabbitMQ 3.7 to 3.8](https://youtu.be/DynCqFtnSoY)
## Ready to learn more?
* 13 Feb, online: Free webinar on How to [Build Reliable Streaming Pipelines with RabbitMQ and Project Reactor](https://content.pivotal.io/rabbitmq/feb-13-how-to-build-reliable-streaming-pipelines-with-rabbitmq-and-project-reactor-webinar?utm_campaign=reactor-streaming-webinar-blog&utm_source=rabbitmq&utm_medium=website)
* 20 Feb, online: Hashitalks 2020 features a talk on [Securing RabbitMQ with Vault](https://events.hashicorp.com/hashitalks2020) by <NAME> (@devops_rob)
* 28 Feb, online: TGIR S01E02: Help! RabbitMQ ate my RAM!
* 5-6 Mar, San Francisco: [Code BEAM SF](https://codesync.global/conferences/code-beam-sf/) which features these talks on RabbitMQ:
* A Novel Application Of Rabbitmq For The Reliable Automated Deployment Of Software Updates with <NAME> (@brc859844) and <NAME>
* How RabbitMQ simplifies routing in a microservices architecture with <NAME> and <NAME>
* On-demand, online at LearnFly: [Learn RabbitMQ Asynchronous Messaging with Java and Spring](https://www.learnfly.com/learn-rabbitmq-asynchronous-messaging-with-java-and-spring)
* On-demand, online at Udemy: [RabbitMQ: Messaging with Java, Spring Boot And Spring MVC](https://www.udemy.com/rabbitmq-messaging-with-java-spring-boot-and-spring-mvc/)
* Online, $40 buys you early access to Marco Behler’s course, [Building a Real-World Java and RabbitMQ Messaging Application](https://www.marcobehler.com/courses/30-building-a-real-world-java-and-rabbitmq-messaging-amqp-application)
* Online, Pluralsight course: [RabbitMQ by Example](https://www.pluralsight.com/courses/rabbitmq-by-example) gets good reviews
* Online: Luxoft is offering a [RabbitMQ course in Russian](https://www.luxoft-training.ru/kurs/platforma_obmena_soobshcheniyami_rabbitmq.html)
* Various: South Africa: [Jumping Bean offers RabbitMQ training](https://www.jumpingbean.co.za/rabbitmq)
<file_sep>---
id: point-to-point
title: Point-to-Point
---
TODO<file_sep>---
id: configure-queue-length-limit-policy
title: Configure queue length limit policy
---
Beside the configuration settings you discovered in the previous step,
you can use policies to fine-tune the behavior of RabbitMQ, at runtime
and on a per-queue basis for instance.
We will take the example of configuring a length limit to a queue for
this demonstration.
> **TODO**: Write this page.
<file_sep>---
id: build
title: Build RabbitMQ
---
> **TODO**: Write this page.
<file_sep>---
id: streaming-across-clusters
title: Streaming Across Clusters
---
RabbitMQ offers multiple features for streaming messages between separate instances or clusters. There are multiple usecases for cross-cluster streaming that RabbitMQ support natively.
## Cases for Cross-Cluster Streaming
### Edge computing
RabbitMQ instances or clusters can be deployed to edge locations to provide both low-latency messaging for local systems and a routing backbone that routes or replicates messages to a centralised cluster in a data center.

### Peer-to-Peer Clusters
Peer clusters can route messages to each other to allow for message processing on both sides.

### Hot Standby
Messages can be streamed from an active cluster to a standby cluster so that in the event of a disaster, applications be be switched over to the hot-standby.
### Blue/Green Deployments
Consumers and publishers can be migrated from one cluster to another without message loss by streaming messages from the existing primary cluster to a new cluster that will soon become the primary.
### Consumer Load Distribution
Messages of a single queue can be distributed via streaming across counterpart queues on multiple clusters so that consumption load is spread out.
## Features
### Federation
Federation allows for the streaming of messages across multiple RabbitMQ instances or clusters. It is different to replicated queues (Quorum/Classic HA queues) in that it can link separate clusters across a WAN that would not be viable for a replicated queue. Clusters can be of different Erlang/RabbitMQ versions.
There are two type of federation:
- exchange federation
- queue federation
The two satisfy different use cases but both rely on concept of linking two RabbitMQ clusters together.
#### Upstream and Downstream clusters
Federation involves linking two or more clusters into an upstream-downstream relationship. Messages are published to an upstream cluster and those messages can be routed to local queues on the upstream as normal and additionally be configured to be streamed to the downstream cluster

These upstream-downstream pairings can be combined to form many type of graph (hub and spoke, DAG, ring etc).

#### Exchange Federation
We can perform message routing to a downstream cluster using federated exchanges.
- Routing option #1 - we want all messages published to exchanges in the upstream, to be routed to the downstream.
- Routing option #2 - we want all messages published to a particular exchange in the upstream, to be routed to the downstream.
- Routing option #3 - we want to route only a subset of messages published to a particular exchange(s) based on things like routing keys and headers.
A federated exchange can be a fanout, topic, direct or header exchange just like a normal exchange and we can express routing logic across clusters in the usual way we do so within a single cluster.

The normal usecases are:
- Hub and Spoke pattern. Stream messages from multiple regional clusters to a central cluster where you want to process some particular type of message only or have some central auditing.
- Edge/Data Center pattern. Local RabbitMQ clusters serve local systems in an edge location and benefit from low latency. Those messages are in turn (selectively) streamed to clusters in either regional datacenters or a single global data center, when there is connectivity.
- Peer Clusters pattern. Messages published to two regional clusters and must be processed on both clusters. Each cluster plays the role of upstream and downstream, allowing local consumers to consume both locally published messages and message published to the peer cluster.
#### Queue Federation
Queue federation is not about routing but about distributing messages to be consumed across more than one cluster. It allows us to use the competing consumer pattern on a single queue, but across multiple clusters.
The way it works is that a federated queue is created on the downstream cluster which links it to a queue on the upstream. Messages will be streamed from the upstream queue to the downstream queue as long as there is a consumer on the downstream queue with enough capacity. It is a best effort approach to spread load of a single queue across multiple clusters.

For example:
- 1,2,3,4,5 arrive at upstream
- 1,2,3 are consumed from the upstream queue by a local consumer
- 4,5 are streamed to the downstream queue
- 6,7,8 arrive at the upstream
- 4,5 are consumed on the downstream queue by a local consumer
- 6,7 are consumed from the upstream queue by a local consumer
- 8 is streamed to the downstream queue
- 8 is consumed from the downstream queue by a local consumer
The normal usecases are:
- distribute consumption workload across multiple clusters
- blue-green deployments where consumers need to be migrated to a new cluster and not process the same message twice.
#### Federation Topologies
We can create federation topologies where one downstream cluster is the upstream of another. We can form Hub and Spoke, Directed Acyclic Graphs (DAG), rings or whatever you want. Typically, it is recommended to keep federation topologies simple.
### Shovel
Shovels can be used to stream messages from one cluster to another, or one vhost to another.
A shovel is an Erlang process that will consume messages from an exchange or queue on one cluster, and publish them to an exchange or queue on another cluster. It is basically, a well written consumer/publisher application that is hosted within the cluster and uses RabbitMQ's reliable messaging features to provide an At-Least-Once delivery guarantee of streamed messages.
The shovel processes will start-up when the cluster starts and can survive the loss of a broker, performing fail-over to another broker.

It has similarities with Exchange Federation but with some key differences:
- Nomenclature changes from upstream/downstream to source/destination.
- Supports AMQP 1.0. In fact the source could be AMQP 0.9.1 and the destination be AMQP 1.0 or vice-versa.
- Supports different streaming sources/destinations:
- source exchange -> destination exchange
- source queue -> destination exchange
- source exchange -> destination queue
- source queue -> destination queue
- Can be used to stream messages between vhosts on the same cluster
Often both Exchange Federation and Shovel can be a candidate for the same usecase.<file_sep>---
id: pub-sub-fanout
title: Publish-Subscribe with the Fanout exchange
sidebar_label: Pub-Sub with Fanout
---
TODO<file_sep>---
id: confirms
title: Publisher Confirms
---
Ensuring data safety is a collective responsibility of applications, client libraries and RabbitMQ cluster nodes. Messages can be lost in-flight between a publisher and broker.
A message could be lost:
- while in an outgoing or incoming buffer
- while being transmitted over the wire
- while being written to disk
- while being processed by the channel
Publisher confirms are an acknowledgement mechansim that offers At-Least-Once guarantees.
## Basic.ack, basic.nack, basic.return
Confirms come is as either:
- basic.ack: the message has been safely stored by the broker
- basic.nack: some kind of failure occurred and the message has not been safely stored by the broker
- basic.return: the message was unroutable (see the [Unroutable section](#mandatory-flag))
## Strategies for Using Publisher Confirms
Publisher confirms provide a mechanism for application developers to keep track of what messages have been successfully accepted by RabbitMQ. There are several commonly used strategies for using publisher confirms:
- **Streaming**: publish messages continuously, and process incoming confirms continuously. Limiting the number of unconfirmed messages (aka in-flight messages) to predetermined amount.
- **Batching**: publish a batch of messages at a time and wait for all outstanding confirms before sending the next batch.
- **Publish-and-wait**: publish one message at a time, waiting for the confirm before sending the next. This option is **highly** discouraged due to its highly negative impact on publisher throughput.
### Streaming Confirms
Most client libraries usually provide a way for developers to handle individual confirmations as they arrive from the server. The confirms will arrive asynchronously. Since publishing is also inherently asynchronous in AMQP 0-9-1, this option allows for safe publishing with very little overhead. The algorithm is usually similar to this:
- Enable publisher confirms on a channel
- For every published message, add a map entry that maps current sequence number to the message
- When a positive ack arrives, remove the entry
- When a negative ack arrives, remove the entry and schedule its message for republishing (or something else that's suitable)
In the RabbitMQ Java client, confirm handler is exposed via the ConfirmCallback and ConfirmListener interfaces. One or more listeners have to be added to a channel.
### Batch Publishing
This strategy involves publishing batches of messages and awaiting for the entire batch to be confirmed. Retries are performed on batches.
- Enable publisher confirms on a channel
- Send messages of a batch one after the other until all have been sent.
- Wait for all outstanding confirms
- When all confirms come in positive, publish the next batch
- If there are negative confirms or timeout hits, republish the entire batch or only the relevant messages
Some clients provide convenience API elements for waiting for all outstanding confirms. For example, in the Java client there is Channel#waitForConfirms(timeout).
Since this approach involves waiting for confirms, it will have negative effects on publisher throughput. The larger the batch, the smaller the effect will be.
### Publish-and-Wait
This strategy can be considered an anti-pattern and is documented primarily for completeness. It involves publishing a message and immediately waiting for the outstanding acknowledgement to arrive.
This approach will have a very significant negative effect on throughput and is not recommended.
## Mandatory flag
A publisher can request to be notified if a published message was not routed to any queues. It does this by setting the the `mandatory` flag to true. If the message cannot be routed to any queue then the publisher is warned via an additional confirm message called basic.return which is sent to the publisher after a basic.ack.
The possible causes of an unroutable message are:
- message sent to the default exchange but no queue matches the routing key
- message sent to an exchange with no bindings
- message sent to an exchange with bindings, but no bindings match that particular message.<file_sep>---
id: reliable-messaging
title: Reliable Messaging
---
Reliable messaging is about strong guarantees that published messages get delivered to consumers in the face of failures such as TCP connection failures and even the loss of a broker.
There is a chain of responsibility from publisher to broker to consumer and both RabbitMQ and the clients need to behave correctly in order to achieve reliability.
## Guarantees
With messaging systems we tend to talk about either At-Most-Once or At-Least-Once processing guarantees. Additionally we care about ordering guarantees.
### At-Most-Once Guarantee
The At-Most-Once guarantee says that messages might get lost, but a message will never get processed more than once. RabbitMQ offers this guarantee when:
- publishers do not use Publisher Confirms
- consumers use Auto Acknowledgement mode
- messages are stored in a classic queue with a non-redundant storage device
This is a best effort, highest performance option that is good when you don't care if some messages get lost but do care about low latency and high throughput.
### At-Least-Once Guarantee
The At-Least-Once guarantee says that no message will get lost, but might end up being processed by a consumer more than once. We attain this guarantee by ensuring:
- publishers use Publisher Confirms
- consumers use manual consumer acknowedgements correctly
- messages are replicated across multiple brokers or durable, fault tolerant storage is used.
> RAID arrays or cloud block storage (like EBS on AWS) offer replication at the storage layer.
This guarantee offers safety first and usually results in lower throughput and higher latencies.
## Publisher Confirms
In order to not lose a message in-transit between a publisher and a broker, we use an acknowledgement mechansim called Publisher Confirms. Once a broker has safely written a message to disk (and possibly replicated), it will notify the publisher that it now has taken responsibility for that message.
Until this confirm has been received by the publisher, it cannot assume that the message is safe and remains the publisher's responsibility.
## Consumer Acknowledgements
In order not to lose a message in-transit between a broker and a consumer, we use Consumer Acknowledgements. If we want At-Least-Once processing guarantees, the consumer should send an acknowledgement **after** it has safely completed processing the message.
If there is an error while processing the message, then the message can be redelivered for another attempt at processing it.
If the consumer acknowledges a message on receipt, but before processing it, then an error during processing will essentially cause the message to have been lost. It will not be redelivered and will end up having never been successfully processed.
## Replicated Queues
Both Classic HA queues and Quorum queues offer replication of messages over multiple brokers so that in the event of the loss of a broker, no confirm messages are lost and the queue remains operational.
## Durable, Fault Tolerant Storage
Using a storage device that provides redundancy in the case of failure is also a good option. It does not offer high availability by itself as the loss of the broker will cause all hosted queues to be offline. But it can provide data safety.
RAID arrays and cloud block storage are both good options.<file_sep>---
id: integrations
title: Integrations
---
TODO<file_sep>---
id: index
title: Enterprise Edition
---
TODO<file_sep>---
id: troubleshooting
title: Publisher Troubleshooting
sidebar_label: Troubleshooting
---
This section covers a number of common issues with publishers, how to identify and address them. Failures in distributed systems come in many shapes and forms, so this list is by no means extensive.
## Connectivity Failures
Like any client, a publisher has to successfully connect and successfully authenticate first.
The number of potential connectivity issues is pretty broad and has a dedicated guide.
## Authentication and Authorisation
Like any client, a publisher can fail to authenticate or don't have the permissions to access their target virtual host, or publish to the target exchange.
Such failures are logged by RabbitMQ as errors.
See the sections on troubleshooting of authentication and authorisation in the Access Control guide.
## Connection Churn
Some applications open a new connection for every message published. This is highly inefficient and not how messaging protocols were designed to be used. Such condition can be detected using connection metrics.
Prefer long lived connections when possible.
## Connection Interruption
Network connections can fail. Some client libraries support automatic connection and topology recovery, others make it easy to implement connection recovery in application code.
When connection is down, no publishes will go through or be internally enqueued (delayed) by clients. In addition, messages that were previously serialised and written to the socket are not guaranteed to reach the target node. It is therefore critically important for publishers that need reliable publishing and data safety to use Publisher Confirms to keep track of what publishes were confirmed by RabbitMQ. Messages that were not confirmed should be considered undelivered after a period of time. Those messages can be republished if it's safe to do so for the application. This is covered in tutorial 7 and the Data Safety section in this guide.
See Recovery from Network Connection Failures for details.
## Routing Issues
A publisher can be successfully connected, authenticated and granted the permissions to publish to an exchange (topic, destination). However, it is possible that such messages would not be routed to any queues or consumers. This can be due to
- A configuration mismatch between applications, e.g. topics used by the publishers and consumers do not match
- Publisher misconfiguration (exchange, topic, routing key are not what they should be)
- For AMQP 0-9-1, missing bindings on the target exchange
- A resource alarm is in effect: see the section below
- Network connection has failed and the client did not recover: see the section above
Inspecting the topology and metrics usually helps narrow the problem quickly. For example, the individual exchange page in management UI can be used to confirm that there is inbound message activity (ingress rate above zero) and what the bindings are.
In the following example the exchange has no bindings, so no messages will be routed anywhere:
An exchange without bindings
Bindings can also be listed using rabbitmq-diagnostics:
```
# note that the implicit default exchange bindings won't
# be listed as of RabbitMQ 3.8
rabbitmq-diagnostics list_bindings --vhost "/"
=> Listing bindings for vhost /...
In the example above the command yields no results.
```
Starting with RabbitMQ 3.8, there's a new metric for unroutable dropped messages:
Unroutable message metrics diagram
In the example above, all published messages are dropped as unroutable (and non-mandatory). See the Unroutable Message Handling section in this guide.
Cluster-wide and connection metrics as well as server logs will help spot a resource alarm in effect.
## Resource Alarms
When a resource alarm is in effect, all connections that publish will be blocked until the alarm clears. Clients can opt-in to receive a notification when they are blocked. Learn more in the Resource Alarms guide.
## Protocol Exceptions
With some protocols, such as AMQP 0-9-1 and STOMP, publishers can run into a condition known as a protocol error (exception). For example, publishing to a non-existent exchange or binding an exchange to an non-existent exchange will result in a channel exception and will render the channel closed. Publishing is not possible on a closed channel. Such events are logged by the RabbitMQ node the publisher was connected to. Failed publishing attempts will also result in client-side exceptions or errors returned, depending on the client library used.
## Concurrent Publishing on a Shared Channel
Concurrent publishing on a shared channel is not supported by client libraries. Learn more in the Concurrency Considerations section.<file_sep>---
id: index
title: The Basics
---
## The Basics
Publishers publish to a destination that varies from protocol to protocol:
- In AMQP 0-9-1, publishers publish messages to exchanges.
- In AMQP 1.0, publishing happens on a link.
- In MQTT, publishers publish to topics.
- STOMP supports a variety of destination types: topics, queues, AMQP 0-9-1 exchanges.
This is covered in more details in the protocol-specific differences section.
A message is sent down a channel established between the publisher and a broker. On the broker side, the channel process will determin the destination queue(s) by looking up the exchange and its bindings. The message will then be forwarded on to any matching queues.
## Publisher Lifecycle
Publishers are often long lived: that is, throughout the lifetime of a publisher it publishes multiple messages. Opening a connection or channel (session) to publish a single message is not optimal.
Publishers usually open their connection(s) during application startup. They often would live as long as their connection or even application runs.
Publishers can be more dynamic and begin publishing in reaction to a system event, stopping when they are no longer necessary. This is common with WebSocket clients used via Web STOMP and Web MQTT plugins, mobile clients and so on.
## Protocol Differences
The process of publishing messages is quite similar in every protocol RabbitMQ supports. All four protocols allow the user to publish a message which has a payload (body) and one or more message properties (headers).
All four protocols also support an acknowledgement mechanism for publishers which allows the publishing application to keep track of the messages that have or haven't been successfully accepted by the broker, and continue publishing the next batch or retry publishing the current one.
The difference typically have more to do with the terminology used than the semantics. Message properties also vary from protocol to protocol.
AMQP 0-9-1
### AMQP 0-9-1
In AMQP 0-9-1, publishing happens on a channel using routing rules determined by an exchange. The broker channel process uses a routing topology set up by defining bindings between one or more queues and the exchange, or source exchange and destination exchange. Successfully routed messages are stored in queues.
The role of each entity is covered in the AMQP 0-9-1 concepts guide.
### AMQP 1.0
In AMQP 1.0 publishing happens within a context of a link.
### MQTT 3.1
In MQTT 3.1.1, messages are published on a connection to a topic. Topics perform both routing and storage. In RabbitMQ, a topic is backed by a queue internally.
When publisher chooses to use QoS 1, published messages are acknowledged by the routing node using a PUBACK frame, the publisher acknowledgement mechanism in MQTT 3.1.
Publishers can provide a hint to the server that the published message on the topic must be retained (stored for future delivery to new subscribers). Only the latest published message for each topic can be retained.
Other than closing the connection, there is no mechanism by which the server can communicate a publishing error to the client.
See the MQTT and MQTT-over-WebSockets guides to learn more.
### STOMP
STOMP clients publish on a connection to one or more destinations which can have different semantics in case of RabbitMQ.
STOMP provides a way for the server to communicate an error in message processing back to the publisher. Its variation of publisher acknowledgements is called receipts, which is a feature clients enable when publishing.
See the STOMP guide, STOMP-over-WebSockets and the STOMP 1.2 spec to learn more.<file_sep>---
id: index
title: Exchange Types
---
## Exchange Types
### Default Exchange
### Fanout Exchange
### Direct Exchange
### Topic Exchange
### Header Exchange
### Other Exchange Types
## Exchange Arguments
### Auto-Delete<file_sep>---
id: docker
title: Run using Docker
sidebar_label: Docker
---
> **TODO**: Write this page.
<file_sep>---
id: precompiled
title: Run from precompiled binaries
sidebar_label: Precompiled binaries
---
We provide precompiled binaries for both Unix-like systems and Microsoft
Windows.
## Get Erlang/OTP
RabbitMQ relies on the Erlang/OTP runtime to run.
Please refer to the [Erlang/OTP requirements and install guide](erlang)
to learn about:
* what version of Erlang/OTP to get
* how to install it
## Get and run RabbitMQ
Once the appropriate version of Erlang/OTP is available on your
operating system, it is very easy to run RabbitMQ as an unprivileged
user to play with it.
### Unix-like systems
1. Download the [`generic-unix` archive]:
```sh
wget https://github.com/rabbitmq/rabbitmq-server/releases/download/v3.8.2/rabbitmq-server-generic-unix-3.8.2.tar.xz
```
2. Unpack the archive:
```sh
tar xf rabbitmq-server-generic-unix-3.8.2.tar.xz
```
3. Start RabbitMQ:
```sh
./rabbitmq_server-3.8.2/sbin/rabbitmq-server
```
RabbitMQ will print the following banner:
```text
## ## RabbitMQ 3.8.2
## ##
########## Copyright (c) 2007-2019 Pivotal Software, Inc.
###### ##
########## Licensed under the MPL 1.1. Website: https://rabbitmq.com
Doc guides: https://rabbitmq.com/documentation.html
Support: https://rabbitmq.com/contact.html
Tutorials: https://rabbitmq.com/getstarted.html
Monitoring: https://rabbitmq.com/monitoring.html
Logs: .../rabbitmq_server-3.8.2/var/log/rabbitmq/[email protected]
.../rabbitmq_server-3.8.2/var/log/rabbitmq/rabbit@cassini_upgrade.log
Config file(s): (none)
Starting broker... completed with 0 plugins.
```
[`generic-unix` archive]: https://github.com/rabbitmq/rabbitmq-server/releases/download/v3.8.2/rabbitmq-server-generic-unix-3.8.2.tar.xz
### Microsoft Windows
> **TODO**: Write this section.
<file_sep>---
id: macos
title: Install on macOS
sidebar_label: macOS
---
> **TODO**: Write this page.
<file_sep>---
id: index
title: Welcome to the Developer Reference
sidebar_label: Introduction
---
TODO<file_sep>---
id: index
title: Queues
---
## Basics
## Queue Properties<file_sep>---
id: dynamic-shovels
title: Dynamic Shovels
---
TODO<file_sep>---
id: sharding
title: Sharding / Hash Based Routing
---
TODO<file_sep>---
title: Simplifying rolling upgrades with feature flags
author: <NAME>
---
In this post we will cover **<a href="https://next.rabbitmq.com/feature-flags.html">feature flags</a>**, a new subsystem in RabbitMQ 3.8. Feature flags will allow a rolling cluster upgrade to the next minor version, without requiring all nodes to be stopped before upgrading.
<!--truncate-->
## Minor Version Upgrades Today: RabbitMQ 3.6.x to 3.7.x
It you had to upgrade a cluster from RabbitMQ 3.6.x to 3.7.x, you probably had to use one of the following solutions:
* Deploy a new cluster alongside the existing one (this strategy is known as the [blue-green deployment](blue-green-deployment.html)), then migrate data & clients to the new cluster
* Stop all nodes in the existing cluster, upgrade the last node that was stopped first, then continue upgrading all other nodes, one-by-one
Blue-green deployment strategy is low risk but also fairly complex to automate. On the other hand, a cluster-wide shutdown affects availability. Feature flags are meant to provide a 3rd option by making rolling cluster upgrades possible and reasonably easy to automate.
## The Feature Flags Subsystem
Feature flags indicate a RabbitMQ node's capabilities to its cluster peers. Previously nodes used versions to assess compatibility with cluster versions. There are many ways in which nodes in a distributed system can become incompatible, including Erlang and dependency versions. Many of those aspects are not reflected in a set of version numbers. Feature flags is a better approach as it can reflect more capabilities of a node, whether it is a particular feature or internal communication protocol revision. In fact, with some message protocols RabbitMQ supports clients have a mechanism for clients to indicate their capabilities. This allows client libraries to evolve and be upgraded independently of RabbitMQ nodes.
For example, RabbitMQ 3.8.0 introduces a new queue type, <a href="https://next.rabbitmq.com/quorum-queues.html">quorum queues</a>. To implement them, an internal data structure and a database schema were modified. This impacts the communication with other nodes because the data structure is exchanged between nodes, and internal data store schema is replicated to all nodes.
Without the feature flags subsystem, it would be impossible to have a RabbitMQ 3.8.0 node inside a cluster where other nodes are running RabbitMQ 3.7.x. Indeed, the 3.7.x nodes would be unable to understand the data structure or the database schema from 3.8.0 node. The opposite is also true. That's why RabbitMQ today prevents this from happening by comparing versions and by denying clustering when versions are considered incompatible (the policy considers different minor/major versions to be incompatible).
New in RabbitMQ 3.8.0 is the feature flags subsystem: when a single node in a 3.7.x cluster is upgraded to 3.8.0 and restarted, it will not immediately enable the new features or migrate its database schema because the feature flags subsystem told it not to. It could determine this because RabbitMQ 3.7.x supports no feature flags at all, therefore new features or behaviours in RabbitMQ 3.8.0 cannot be used before all nodes in the cluster are upgraded.
After a partial upgrade of a cluster to RabbitMQ 3.8.0, all nodes are acting as 3.7.x nodes with regards to incompatible features, even the 3.8.0 one. In this situation, quorum queues are unavailable. Operator must finish the upgrade by upgrading all nodes. When that is done, the operator can decide to enable the new feature flags provided by RabbitMQ 3.8.0: one of them enables quorum queues. This is done using RabbitMQ CLI tools or <a href="https://www.rabbitmq.com/management.html">management UI</a> and supposed to be performed by deployment automation tools. The idea is that the operator needs to confirm that her cluster doesn't have any remaining RabbitMQ 3.7.x nodes that might rejoin the cluster at a later point.
Once a new feature flag is enabled, it is impossible to add a node that runs an older version to that cluster.
## Demo with RabbitMQ 3.8.0
Let's go through a complete upgrade of a RabbitMQ 3.7.x cluster. We will take a look at the feature flags in the process.
We have the following 2-node cluster running RabbitMQ 3.7.12:
<img style="border: solid 1px #75757f;" src="https://www.rabbitmq.com/img/blog/feature-flags/01-list-of-nodes-on-node-A-running-3.7.x.png" alt="" />
We now upgrade node A to RabbitMQ 3.8.0 and restart it. Here is what the management overview page looks like after the node is restarted:
<img style="border: solid 1px #75757f;" src="https://www.rabbitmq.com/img/blog/feature-flags/02-list-of-nodes-on-node-A-running-3.8.x.png" alt="" />
We can see the difference of versions in the list of nodes: their version is displayed just below their node name.
The list of feature flags provided by RabbitMQ 3.8.0 is now available in the management UI on node A:
<img style="border: solid 1px #75757f;" src="https://www.rabbitmq.com/img/blog/feature-flags/03-list-of-feature-flags-on-node-A-running-3.8.x.png" alt="" />
This page will not exist on node B because it is still running RabbitMQ 3.7.12.
On node A, we see that the `quorum_queue` feature flag is marked as `Unavailable`. The reason is that node B (still running RabbitMQ 3.7.12) does not known about `quorum_queue` feature flag, therefore node A is not allowed to use that new feature flag. This feature flag cannot be enabled until all nodes in the cluster support it.
For instance, we could try to declare a quorum queue on node A, but it is denied:
<img style="border: solid 1px #75757f;" src="https://www.rabbitmq.com/img/blog/feature-flags/04-quorum-queue-declare-denied-on-node-A-running-3.8.x.png" alt="" />
After node B is upgraded, feature flags are available and they can be enabled. We proceed and enable `quorum_queue` by clicking the `Enable` button:
<img style="border: solid 1px #75757f;" src="https://www.rabbitmq.com/img/blog/feature-flags/05-quorum_queue-feature-flag-enabled-on-node-B-running-3.8.x.png" alt="" />
Now, we can declare a quorum queue:
<img style="border: solid 1px #75757f;" src="https://www.rabbitmq.com/img/blog/feature-flags/06-quorum-queue-declare-accepted-on-node-A-running-3.8.x.png" alt="" />
## To Learn More
The <a href="https://next.rabbitmq.com/feature-flags.html">Feature Flags subsystem documentation</a> describes in greater details how it works and what operators and plugin developers should pay attention to.
Note that feature flags are not a guarantee that a cluster shutdown will never be required for upgrades in the future: the ability to implement a change using a feature flag depends on the nature of the change, and is decided on a case-by-case basis. Correct behaviour of a distributed system may require that all of its components behave in a certain way, and sometimes that means that they have to be upgraded in lockstep.
Please give feature flags a try and let us know what you think on the <a href="https://groups.google.com/forum/#!forum/rabbitmq-users">RabbitMQ mailing list</a>!
<file_sep>---
title: RabbitMQ 3.8.2 release
author: <NAME>
---
The RabbitMQ team is pleased to announce the release of RabbitMQ 3.8.2.
This is a maintenance release. Release notes can be found in the [change
log](/changelog.html). RabbitMQ 3.7 series will [go out of support on
March 31, 2020](/versions.html).
This release [requires Erlang/OTP 21.3](/which-erlang.html).
Binary builds and packages of the new release can be found on
[GitHub](https://github.com/rabbitmq/rabbitmq-server/releases/),
[Package Cloud](https://packagecloud.io/rabbitmq) and
[Bintray](https://bintray.com/rabbitmq/).
See [RabbitMQ downloads](/download.html) page for installation guides.
We encourage all users of earlier versions of RabbitMQ to upgrade to
this latest release.
As always, we welcome any questions, bug reports, and other
feedback on this release, as well as general suggestions for
features and enhancements in future releases. Contact us via the
[rabbitmq-users](https://groups.google.com/forum/#!forum/rabbitmq-users)
Google group.
<file_sep>---
id: throttling
title: Publisher Throttling and Resource Alarms
sidebar_label: Publisher Throttling
---
When a cluster node has a [resource alarm](?) in effect, all connections in the cluster that attempt to publish a message will be blocked until all alarms across the cluster clear.
When a connection is blocked, no more data sent by this connection will be read, parsed or processed on the connection. When a connection is unblocked, all client traffic processing resumes.
Compatible AMQP 0-9-1 clients will be notified when they are blocked and unblocked.
Writes on a blocked connection will time out or fail with an I/O write exception.<file_sep>---
id: debian
title: Install on Debian and Ubuntu
sidebar_label: Debian
---
## Provide Erlang/OTP
Erlang/OTP packages in the official Debian and Ubuntu repositories are
often too old for RabbitMQ's [Erlang/OTP version requirements](erlang).
There are several providers of recent Erlang/OTP releases. Each one has
its own section.
If you already have Erlang available as a Debian package, you can [skip
to the next section](#install-rabbitmq).
### RabbitMQ repository [recommended]
The RabbitMQ team provides its own Erlang/OTP packages for Debian &
Ubuntu. This is the recommended repository.
#### Configure the repository
1. Add the repository to the sources list after replacing
[`$distribution`](#erlangotp-supported-debian-and-ubuntu-distributions)
and [`$component`](#erlangotp-repository-components-available):
```sh
sudo cat <EOF >/etc/apt/sources.list.d/rabbitmq-erlang.list
deb http://dl.bintray.com/rabbitmq-erlang/debian $distribution $component
EOF
```
2. Install `apt-transport-https` to allow apt-get(8) to use the added
repository:
```sh
sudo apt-get install apt-transport-https
```
3. Add the [PGP key used to sign the repository][PGP signing key]:
```sh
wget -O- https://github.com/rabbitmq/signing-keys/releases/download/2.0/rabbitmq-release-signing-key.asc | \
sudo apt-key add -
```
4. Refresh the APT package index:
```sh
sudo apt-get update
```
### Erlang Solutions
> **TODO**: Write this section
## Install RabbitMQ
1. Add the repository to the sources list after replacing
[`$distribution`](#supported-debian-and-ubuntu-distributions)
and [`$component`](#rabbitmq-repository-components):
```sh
sudo cat <EOF >/etc/apt/sources.list.d/rabbitmq-erlang.list
deb http://dl.bintray.com/rabbitmq/debian $distribution $component
EOF
```
2. Install `apt-transport-https` to allow apt-get(8) to use the added
repository:
```sh
sudo apt-get install apt-transport-https
```
3. Add the [PGP key used to sign the repository][PGP signing key]:
```sh
wget -O- https://github.com/rabbitmq/signing-keys/releases/download/2.0/rabbitmq-release-signing-key.asc | \
sudo apt-key add -
```
4. Refresh the APT package index:
```sh
sudo apt-get update
```
5. Install the `rabbitmq-server` package:
```sh
sudo apt-get install rabbitmq-server
```
## Start RabbitMQ
As per de Debian Policy, RabbitMQ is started automatically when the
package is installed.
Depending on the version of Debian/Ubuntu you use, you can start,
restart and stop it using either SysV init scripts or systemd.
### Start service with systemd
```sh
systemctl start rabbitmq-server
systemctl restart rabbitmq-server
systemctl stop rabbitmq-server
```
### Start service with SysV init script
```sh
service rabbitmq-server start
service rabbitmq-server restart
service rabbitmq-server stop
```
## Supported Debian and Ubuntu distributions
The repository supports several distributions of Debian and Ubuntu:
| Distribution| Value of `$distribution` in the sources.list |
|-----------------------|-----------|
| Debian Jessie (8) | `jessie` |
| Debian Stretch (9) | `stretch` |
| Debian Buster (10) | `buster` |
| Debian Sid (unstable) | `buster` |
| Ubuntu 16.04 | `xenial` |
| Ubuntu 18.04 | `bionic` |
| Ubuntu 19.04 | `bionic` |
| Ubuntu 19.10 | `bionic` |
## Repository components available
### RabbitMQ repository components
The repository uses components to allow to "pin" to a RabbitMQ release
series without pininng a specific version.
The following components are available:
| Component | What it provides |
|-----------|------------------|
| `main` | All packages |
| `rabbitmq-server` | All `rabbitmq-server` packages |
| `rabbitmq-server-v3.6.x` | All `rabbitmq-server` 3.6.x packages |
| `rabbitmq-server-v3.7.x` | All `rabbitmq-server` 3.7.x packages |
| `rabbitmq-server-v3.8.x` | All `rabbitmq-server` 3.8.x packages |
### Erlang/OTP repository components
The repository uses components to allow to "pin" to an Erlang/OTP
release series without pininng a specific version.
The following components are available:
| Component | What it provides |
|-----------|------------------|
| `main` | All versions of the Erlang/OTP and Elixir packages |
| `erlang` | All versions of the Erlang/OTP packages |
| `erlang-19.x` | All Erlang/OTP 19.x packages |
| `erlang-20.x` | All Erlang/OTP 20.x packages |
| `erlang-21.x` | All Erlang/OTP 21.x packages |
| `erlang-22.x` | All Erlang/OTP 22.x packages |
[PGP signing key]: https://github.com/rabbitmq/signing-keys/releases/download/2.0/rabbitmq-release-signing-key.asc
<file_sep>---
id: windows
title: Install on Microsoft Windows
sidebar_label: Microsoft Windows
---
> **TODO**: Write this page.
<file_sep>---
id: avoiding-overload
title: Avoiding Overload
---
Talk about features such as queue limit policies.
TODO<file_sep>---
id: federated-queues
title: Federated Queues
---
TODO<file_sep>---
id: consume-message
title: Consume a message
---
In the previous step, we published a message after writing a small
Python AMQP 0-9-1 program. Our next goal is to consume that message
using another Python program.
> **TODO**: Write this page.
<file_sep>---
id: safety-at-rest
title: Safety At-Rest
---
TODO<file_sep>---
id: use-perftest
title: Run PerfTest
---
When you want to quickly test a RabbitMQ node or cluster, you can use
PerfTest to easily publish and consume arbitrary messages without having
to develop your own client.
> **TODO**: Write this page.
<file_sep>---
id: index
title: Introduction
---
One reason for choosing RabbitMQ is its powerful routing capability. In this set of guides will look at some archetypical routing scenarios and some more creative examples that demonstrate what you can do with RabbitMQ.
1. Point-to-point
2. Publish-subscribe with fanout exchange
3. Publish-subscribe with topic, direct, header exchanges
4. Multi-Layered Routing
5. Public Message Exchange, Private Consumer Exchange
6. Sharding / Hash Based Routing
7. Ephemeral infrastructure
8. Class of Service / Priority Routing
9. RPC / Reply To Queues
10. Delayed Message Routing
11. Retry Message Routing<file_sep>---
id: quorum-queues
title: Quorum Queues
---
TODO<file_sep>---
id: query-queue-infos
title: Query queue informations
---
The previous steps allowed us to publish to and consume messages from
RabbitMQ, using two independent programs.
Now that messages are flowing through RabbitMQ, it is important to
observe and monitor it to ensure everything is healthy. The first tool
we will explore is the CLI: we will use it to query various informations
of a queue.
> **TODO**: Write this page.
<file_sep>---
id: multi-layered-routing
title: Multi-Layered Routing
---
TODO<file_sep>---
title: Moving website to Docusaurus
author: <NAME>
---
We finally moved from a home-grown website almost-static engine to an
open-source generator called [Docusaurus](https://docusaurus.io/).
We are happy with the change!
It even supports Erlang hilighting:
```erlang
hello_world() ->
io:format("Hello World!~n").
```
<file_sep>---
id: index
title: Consumers
---
## Basics
<file_sep>---
id: stream-processing
title: Stream Processing Frameworks
---
TODO<file_sep>---
id: intro
title: Introduction to RabbitMQ
---
## Messaging that just works
RabbitMQ is an open source messaging system that supports a variety of messaging use cases including:
- Microservice asynchronous communication
- Publish/Subscribe
- Point-to-point communication
- Work queues
- Request/Reply mechanism without the need for service discovery
- IOT communications via MQTT
- Web frontend-backend communication via STOMP
- High availability
See our [Concepts](concepts/index.md) page to learn more about general messaging patterns and the RabbitMQ building blocks that enable you to build the messaging architecture that you need.
## Why RabbitMQ?
There are many choices out there, so what makes RabbitMQ a great choice?
### Simple to deploy, simple to run.
Unlike many other messaging systems, RabbitMQ is a single, integrated system, without dependencies on external consensus services such as Etcd or Apache ZooKeeper. It is not complex to operate and does not require a dedicated operations teamto run it.
It deploys on all major Linux distributions, Windows and has first class Kubernetes support.
### Supports many protocols, many languages and use cases
RabbitMQ natively supports AMQP 0.9.1 but also supports:
- AMQP 1.0
- MQTT
- STOMP
It has client libraries in most programming languages.
### Integrated UI out-of-the-box and integrations with Grafana and Prometheus
RabbitMQ has a web user interface and API out-of-the-box simplying usage. You don't need to rely on third party web consoles or be forced to use the CLI tooling.
### Extremely flexible, supporting architectures that evolve
They call RabbitMQ the Swiss army knife of messaging. It supports many use cases meaning you don't need to deploy multiple messaging solutions to support your architecture. As your architecture changes, RabbitMQ is flexible enough to support your changing needs.
### Battle tested
RabbitMQ has been used in production for over 10 years and is one of the most widely deployed messaging systems in the world today.
### Open Source
RabbitMQ is open source and we welcome contributions. Please check out our [Be a Contributor](contribution/index.md) page to learn more.
We also have various ways for the community to engage:
- [RabbitMQ Users Google Group aka the mailing list](https://groups.google.com/forum/#!forum/rabbitmq-users)
- [GitHub Discussions](https://github.com/rabbitmq/discussions)
- [Slack channel](https://rabbitmq-slack.herokuapp.com/)
### Commercial Support
For those that need it, we offer commericial support and also an enterprise version of RabbitMQ which ships with extra features important for the enterprise.
<file_sep>---
id: alternate-exchange
title: Alternate Exchanges
---
TODO<file_sep>---
id: failures
title: Types of Publisher Failure
---
## Connection failures
Network connection between clients and RabbitMQ nodes can fail. How applications handle such failures directly contributes to the data safety of the overall system.
### Heartbeats
It can take time to detect the loss of a TCP connection so in order to speed up connection loss detection, an explicit [heartbeat](?) can be configured when opening the connection.
### Connection Recovery
Several RabbitMQ clients support automatic recovery of connections and topology (queues, exchanges, bindings, and consumers): Java, .NET, Bunny are some examples.
Other clients do not provide automatic recovery as a feature but do provide examples of how application developers can implement recovery.
The automatic recovery process for many applications follows the following steps:
- Reconnect to a reachable node
- Restore connection listeners
- Restore channel listeners
- Re-open channels
- Restore channel listeners
- Restore channel basic.qos setting, publisher confirms and transaction settings
After connections and channels are recovered, topology recovery can start. Topology recovery includes the following actions, performed for every channel:
- Re-declare exchanges (except for predefined ones)
- Re-declare queues
- Recover all bindings
- Recover all consumers
## Confirm timeouts
When a confirm is not received after a configured time period the publisher must choose whether it should send the mesage again or not. Resending corresponds with At-Least-Once guarantees while not sending could result in the message being lost (at-most-once).
## Non-existent exchange
An attempt to publish to a non-existent exchange will result in a channel-level exception with the code of 404 Not Found and render the channel it was attempted on to be closed.
## Unroutable
If the publisher has set the [mandatory flag](confirms#mandatory-flag) then it will be notified if the message could not be routed to a queue. If the flag is not set then the message is silently dropped.
## Queue Full
A queue can have a [length or size limit](?) applied. When at that limit, a queue will either:
- reject the message (when [reject-publish](?) has been configured).
- accept the message but drop the oldest message (when [drop-head](?) has been configured and no [deadletter exchange](?) has been configured).
- accept the message but eject the oldest message from the queue and forward it to the configured deadletter exchange (when [drop-head](?) has been configured and also a [deadletter exchange](?) has been configured).
## Channel process crash
Channel process crashes are extremely rare and result in the loss of the channel. A new channel must be reopened.
## Blocked Connection (publisher throttling)
When a RabbitMQ broker is being overloaded by publishers, it uses a [flow control mechanism to throttle publishers](throttling). When a connection is blocked, no messages can be sent down any open channels.
## Notes on Exception Handling
Publishers generally can expect two types of exception:
- A network I/O exception due to a failed write or timeout
- An acknowledgement delivery timeout
Note that "exception" here means an error in the general sense; some programming languages do not have exceptions at all so clients there would communicate the error differently. The discussion and recommendations in this section should apply equally to most client libraries and programming languages.
The former type of exception can occur immediately during a write or with a certain delay. This is because certain types of I/O failures (e.g. to high network congestion or packet drop rate) can take time to detect. Publishing can continue after the connection recovers but if the connection is blocked due to an alarm, all further attempts will fail until the alarm clears. This is covered in more details below in the [Publisher Throttling page](throttling).
The latter type of exception can only happen when the application developer provides a timeout. What timeout value is reasonable for a given application is decided by the developer. It should not be lower than the effective heartbeat timeout.<file_sep>---
id: index
title: Policies
---
## Basics
## Setting policies<file_sep>#!/bin/sh
# vim:sw=4:et:
# This is a script to quickly get a running RabbitMQ node as an
# unprivileged user, based on the generic-unix archive.
set -u
LATEST_BRANCH=3.8
main() {
downloader --check
need_cmd mktemp
need_cmd erl
local _ansi_escapes_are_valid=false
if [ -t 2 ]; then
if [ "${TERM+set}" = 'set' ]; then
case "$TERM" in
xterm*|rxvt*|urxvt*|linux*|vt*)
_ansi_escapes_are_valid=true
;;
esac
fi
fi
# 1. Create temporary directory (for download & Erlang build)
local _dir
_dir="$(mktemp -d 2>/dev/null || ensure mktemp -d -t rabbitmq)"
# 2. Query latest RabbitMQ version.
if $_ansi_escapes_are_valid; then
printf "\033[1minfo:\033[0m querying latest ${LATEST_BRANCH}.x version\n" 1>&2
else
printf '%s\n' "info: querying latest ${LATEST_BRANCH}.x version" 1>&2
fi
local _tags_api=https://api.github.com/repos/rabbitmq/rabbitmq-server/git/matching-refs/tags/v${LATEST_BRANCH};
local _tags_list="${_dir}/tags.json"
ensure downloader "$_tags_api" "$_tags_list"
local rmq_version=$(ensure awk '
/"ref":/ {
tag = $0;
sub(/.*\/tags\/v/, "", tag);
sub(/".*$/, "", tag);
if (tag ~ /^[0-9]+\.[0-9]+\.[0-9]+$/) {
latest = tag;
}
}
END {
print latest;
}' "${_tags_list}")
if $_ansi_escapes_are_valid; then
printf "\033[1minfo:\033[0m latest ${LATEST_BRANCH}.x version: ${rmq_version}\n" 1>&2
else
printf '%s\n' "info: latest ${LATEST_BRANCH}.x version: ${rmq_version}" 1>&2
fi
local gen_unix_dir=rabbitmq_server-$rmq_version
local gen_unix_file=rabbitmq-server-generic-unix-$rmq_version.tar.xz
local gen_unix_url=https://github.com/rabbitmq/rabbitmq-server/releases/download/v$rmq_version/$gen_unix_file
local _file="${_dir}/${gen_unix_file}"
local _rmqdir="${_dir}/$gen_unix_dir"
# 3. Download RabbitMQ.
local _url="${gen_unix_url}"
if $_ansi_escapes_are_valid; then
printf "\033[1minfo:\033[0m downloading generic-unix archive\n" 1>&2
else
printf '%s\n' 'info: downloading generic-unix archive' 1>&2
fi
ensure mkdir -p "$_dir"
ensure downloader "$_url" "$_file"
# 4. Unpack RabbitMQ.
if $_ansi_escapes_are_valid; then
printf "\033[1minfo:\033[0m unpacking generic-unix archive\n" 1>&2
else
printf '%s\n' 'info: unpacking generic-unix archive' 1>&2
fi
cd "$_dir"
tar xf "$_file"
# 5. Enable the management plugin.
if $_ansi_escapes_are_valid; then
printf "\033[1minfo:\033[0m enabling management web UI\n" 1>&2
else
printf '%s\n' 'info: enabling management web UI' 1>&2
fi
ensure "$_rmqdir"/sbin/rabbitmq-plugins \
--quiet \
enable \
--offline \
rabbitmq_management >/dev/null
# 6. Start RabbitMQ.
cat <<EOF
,---------------------------------------------------------------------
| RabbitMQ is installed in:
| $_rmqdir
|
| Configuration files:
| $_rmqdir/etc
|
| Log files:
| $_rmqdir/var/log/rabbitmq
|
| Once RabbitMQ has started below:
|
| * You can stop it by typing Ctrl+C in this terminal, or by running:
| $_rmqdir/sbin/rabbitmqctl stop
|
| * You can restart it later by running:
| $_rmqdir/sbin/rabbitmq-server
|
| * You can enable and disable plugins using:
| $_rmqdir/sbin/rabbitmq-plugins
|
| * The management web UI is available at:
| http://localhost:15672
|
| Login: guest
| Password: <PASSWORD>
\`--------------------------------------------------------------------
EOF
if $_ansi_escapes_are_valid; then
printf "\033[1minfo:\033[0m starting RabbitMQ\n" 1>&2
else
printf '%s\n' 'info: starting RabbitMQ' 1>&2
fi
exec "$_rmqdir"/sbin/rabbitmq-server
}
# --------------------------------------------------------------------
# Code taken from Rustup installeri script.
# See:
# https://www.rust-lang.org/learn/get-started
# https://sh.rustup.rs.
# --------------------------------------------------------------------
say() {
printf 'get-rabbitmq: %s\n' "$1"
}
err() {
say "$1" >&2
exit 1
}
need_cmd() {
if ! check_cmd "$1"; then
err "need '$1' (command not found)"
fi
}
check_cmd() {
command -v "$1" > /dev/null 2>&1
}
# Run a command that should never fail. If the command fails execution
# will immediately terminate with an error showing the failing
# command.
ensure() {
if ! "$@"; then err "command failed: $*"; fi
}
# This wraps curl or wget. Try curl first, if not installed,
# use wget instead.
downloader() {
local _dld
if check_cmd curl; then
_dld=curl
elif check_cmd wget; then
_dld=wget
elif check_cmd fetch; then
_dld=fetch
else
_dld='curl, wget or fetch' # to be used in error message of need_cmd
fi
if [ "$1" = --check ]; then
need_cmd "$_dld"
elif [ "$_dld" = curl ]; then
if ! check_help_for curl --proto --tlsv1.2; then
echo "Warning: Not forcing TLS v1.2, this is potentially less secure"
curl --silent --show-error --fail --location "$1" --output "$2"
else
curl --proto '=https' --tlsv1.2 --silent --show-error --fail --location "$1" --output "$2"
fi
elif [ "$_dld" = wget ]; then
if ! check_help_for wget --https-only --secure-protocol; then
echo "Warning: Not forcing TLS v1.2, this is potentially less secure"
wget "$1" -O "$2"
else
wget --https-only --secure-protocol=TLSv1_2 "$1" -O "$2"
fi
elif [ "$_dld" = fetch ]; then
fetch -o "$2" "$1"
else
err "Unknown downloader" # should not reach here
fi
}
check_help_for() {
local _cmd
local _arg
local _ok
_cmd="$1"
_ok="y"
shift
# If we're running on OS-X, older than 10.13, then we always
# fail to find these options to force fallback
if check_cmd sw_vers; then
if [ "$(sw_vers -productVersion | cut -d. -f2)" -lt 13 ]; then
# Older than 10.13
echo "Warning: Detected OS X platform older than 10.13"
_ok="n"
fi
fi
for _arg in "$@"; do
if ! "$_cmd" --help | grep -q -- "$_arg"; then
_ok="n"
fi
done
test "$_ok" = "y"
}
main "$@" || exit 1
<file_sep>---
id: deadletter-exchange
title: Deadletter Exchanges
---
TODO<file_sep>---
id: routing
title: Message Routing
---
RabbitMQ's powerful routing is one of its killer features. Other messaging systems are based on topics where messages go into a topic and out of a topic. Message ordering across topics is not possible.
RabbitMQ has a different model. Publishers and consumers are decoupled so that any consumer can receive any combination of events in temporal order. Publishers simply name an exchange, add some optional meta-data and no more. This flexibility creates evolutionary architectures that are easy to change.
## Messages
A publisher sends a message payload, such as a JSON document, alongwith:
- an exchange name
- an optional routing key (a string value)
- message properties
- message headers
The channel process on the broker routes the message to queues based on the type of exchange and any queue bindings that exist.
> Remember that exchanges and bindings are meta-data, they consistute the logical routing. Under the hood, messages flow from publisher channel to queue to consumer channel.
## Exchange Types
There are five standard exchange types:
- Default exchange (for point-to-point messaging)
- Fanout (broadcast)
- Topic (routing based on wildcard matching of routing key)
- Direct (routing based on exact match of routing key)
- Headers (routing based on message headers)
There are more exchange types that are covered in the Advanced Routing page.
> The topic, direct and headers exchange can be thought of as filters. Every queue binding is evaluated to see if the message should be routed to that queue or filtered out.
### Default Exchange
When no exchange name is given, the assumed exchange is the amq.default exchange. The message is routed to a queue that matches the routing key. This is the only exchange that gives the publisher the power to choose the destination queue.
For example, the eCommerce site sends a command to the Accounts service to create an account.

### Fanout exchange
Use this exchange when you want multiple queues to receive every message without any conditional per-queue filtering. This is classic, simple publish-subscribe.
For example, both the three different services all want to consume all events. Each service has its own queue and each queue is bound to the fanout exchange.

### Topic exchange
This exchange will route messages based on wildcard matching of the routing key. A routing key can be comprised of a single word, or multiple words separated by dots, such as order.placed.
The available wildcards are:
- \* wildcard that spans one word only
- \# wildcard that spans one or more words
For example, we have the following events:
- order.placed
- order.cancelled
- order.billing.complete
- order.billing.failed
- order.shipping.complete
- order.shipping.failed
- account.created
- account.deleted
We can create bindings with the following binding keys:
- order.placed - exact match
- order.*.complete - all messages related to a completed sub process of orders
- \*.shipping.\* - all messages related to shipping
- order.# - all messages related to orders
This allows for publish-subscribe where each subscribing service filters out the messages it doesn't care about.

### Direct exchange
This exchange is a routing rule like the topic exchange except that it only supports exact match on routing keys. It has slightly higher performance than the topic exchange which would be the reason why you might choose it over the more flexible topic exchange.

### Header exchange
This exchange allows for bindings to filter messages according to headers. A binding can specify one or more headers and their match value. Additionally it specifies whether ALL or ANY of the headers must match.
For example, if we have the custom headers:
- department
- service-level
We could specify a match on one, both or either.
- Match department=sales
- Match both department=sales and service-level=gold
- Match either department=sales or service-level=gold
This exchange is useful when you cannot express all routing within a routing key.
<file_sep>---
id: outbox-pattern
title: Outbox Pattern
---
TODO<file_sep>---
id: shovels
title: Shovels
---
TODO<file_sep>---
id: connections
title: Connections and Channels
---
Clients should open long-lived connections to get the best performance out of RabbitMQ. Once a connection is opened, the second step is to open a **channel**. A channel can be thought of as a virtual connection that multiplexes into a single AMQP connection. It allows a client to open multiple virtual connections with low overhead.
Establishing an AMQP connection and channel is expensive and requires many roundtrips, therefore it is highly advisable to create long lived connections and channels. Creating a connection per message published is extremely inefficient and will yield very low throughput.<file_sep>// vim:sw=2:et:
/**
* Copyright (c) 2020-present, Pivotal Software, Inc.
*
* This source code is licensed under the MIT license found in the
* LICENSE file in the root directory of this source tree.
*/
const React = require('react');
const BlogSidebar = require('../../core/BlogSidebar.js');
const Md = require(`${process.cwd()}/core/Md.js`);
class HomeHero extends React.Component {
render() {
return (
<div id='hero'>
<div id='hero-pipeline-webinar' className='container'>
<div className='text'>
<h1>Streaming Pipelines</h1>
<h2>A webinar on using RabbitMQ and Project Reactor</h2>
<h2>Feb 13 | <a href="https://content.pivotal.io/rabbitmq/feb-13-how-to-build-reliable-streaming-pipelines-with-rabbitmq-and-project-reactor-webinar?utm_campaign=reactor-streaming-webinar-banner&utm_source=rabbitmq&utm_medium=website">Register today</a></h2>
</div>
</div>
</div>
);
}
}
class Intro extends React.Component {
render() {
var blogSidebarConfig = this.props.config;
blogSidebarConfig.blogSidebarTitle = {default: 'Updates'};
blogSidebarConfig.blogSidebarCount = 5;
return (
<div id='intro'>
<Md id='description'>
{`
# RabbitMQ is the most widely deployed open source message broker.
With tens of thousands of users, RabbitMQ is one of
the most popular open source message brokers. From
[T-Mobile](https://www.youtube.com/watch?v=1qcTu2QUtrU) to
[Runtastic](https://medium.com/@runtastic/messagebus-handling-dead-letters-in-rabbitmq-using-a-dead-letter-exchange-f070699b952b),
RabbitMQ is used worldwide at small startups and large enterprises.
RabbitMQ is lightweight and easy to deploy on premises and in the
cloud. It supports multiple messaging protocols. RabbitMQ can be
deployed in distributed and federated configurations to meet high-scale,
high-availability requirements.
RabbitMQ runs on many operating systems and cloud environments,
and provides a [wide range of developer tools for most popular
languages](/devtools.html).
See how other people are using RabbitMQ:
<div id="UfEmbeddedHub1501190831892"></div>
<script>window._ufHubConfig = window._ufHubConfig || [];window._ufHubConfig.push({'containers':{'app':'#UfEmbeddedHub1501190831892'},'collection':'453624','openLink':function(url){window.open(url);},'lazyloader':{'itemDisplayLimit':3,'maxTilesPerRow':3,'maxItemsTotal':3},'tileSize':'small','enablePageTracking':false,'baseUrl':'https://content.pivotal.io/','filesUrl':'https://content.cdntwrk.com/','generatedAtUTC':'2017-07-27 21:26:47'});</script>
<script>(function(d,t,u){function load(){var s=d.createElement(t);s.src=u;d.body.appendChild(s);}if(window.addEventListener){window.addEventListener('load',load,false);}else if(window.attachEvent){window.attachEvent('onload',load);}else{window.onload=load;}}(document,'script','https://content.pivotal.io/hubsFront/embed_collection'));</script>
<p id='morelinkafterufembed'><a href='https://content.pivotal.io/rabbitmq'>More <span className="link-arrow"></span></a></p>
`}
</Md>
<div id='news'>
<div id='updates'>
<BlogSidebar
language="en"
config={blogSidebarConfig}
/>
<p><a href='/blog/'>More updates<span className='link-arrow'></span></a></p>
</div>
<div id='twitterfeed'>
<h2>Tweets</h2>
<a className="twitter-timeline" href="https://twitter.com/RabbitMQ" data-chrome="noheader nofooter noborders transparent noscrollbar" data-tweet-limit='2'></a>
<script src="//platform.twitter.com/widgets.js" charSet="utf-8"></script>
<p><a href='https://twitter.com/RabbitMQ'>More tweets<span className='link-arrow'></span></a></p>
</div>
</div>
</div>
);
}
}
class Features extends React.Component {
render() {
return (
<div id='features' className='home-section-container'>
<div className='home-section'>
<h1>RabbitMQ Features</h1>
<div className='tiles'>
<Md className='tile'>
{`

## Asynchronous Messaging
Supports [multiple messaging protocols](/protocols.html),
[message queuing](/tutorials/tutorial-two-python.html),
[delivery acknowledgement](/reliability.html),
[flexible routing to queues](/tutorials/tutorial-four-python.html),
[multiple exchange type](/tutorials/amqp-concepts.html).
`}
</Md>
<Md className='tile'>
{`

## Developer Experience
Deploy with [BOSH, Chef, Docker and Puppet](/docs/install/). Develop
cross-language messaging with favorite programming languages such
as: Java, .NET, PHP, Python, JavaScript, Ruby, Go, [and many
others](/devtools.html).
`}
</Md>
<Md className='tile'>
{`

## Distributed Deployment
Deploy as [clusters](/clustering.html) for high availability and
throughput; [federate](/federation.html) across multiple availability
zones and regions.
`}
</Md>
<Md className='tile'>
{`

## Enterprise & Cloud Ready
Pluggable [authentication](/authentication.html),
[authorisation](/access-control.html) supports [TLS](/ssl.html) and
[LDAP](/ldap.html). Lightweight and easy to deploy in public and private
clouds.
`}
</Md>
<Md className='tile'>
{`

## Tools & Plugins
Diverse array of [tools and plugins](/devtools.html) supporting
continuous integration, operational metrics, and integration to other
enterprise systems. Flexible [plug-in approach](/plugins.html) for
extending RabbitMQ functionality.
`}
</Md>
<Md className='tile'>
{`

## Management & Monitoring
HTTP-API, command line tool, and UI for [managing and
monitoring](/management.html) RabbitMQ.
`}
</Md>
</div>
</div>
</div>
);
}
}
class GetStarted extends React.Component {
render() {
return (
<div id='getstarted' className='home-section-container'>
<div className='home-section'>
<h1>Get Started</h1>
<div className='tiles'>
<div className='tile'>
<a className='btn btn-secondary' href='/docs/install/'>Download + Installation</a>
<p>Servers and clients for popular operating systems and languages</p>
</div>
<div className='tile'>
<a className='btn btn-primary' href='/docs/getting-started/'>RabbitMQ Tutorials</a>
<p>Hands-on examples to get you started with RabbitMQ</p>
</div>
</div>
</div>
</div>
);
}
}
class Support extends React.Component {
render() {
return (
<div id='support' className='home-section-container'>
<div className='home-section'>
<h1>RabbitMQ Commercial Services</h1>
<Md id='commercial-items'>
{`
<img src="/img/commercial/commercial-distribution-phone.svg" id='commercial-distribution-phone' />
## Commercial Distribution
Pivotal Software offers a [range of commercial offerings for
RabbitMQ](https://pivotal.io/rabbitmq).
This includes a distribution called [Pivotal RabbitMQ](https://network.pivotal.io/products/pivotal-rabbitmq),
a version that deploys in [Pivotal Platform](https://pivotal.io/platform/services-marketplace/messaging-and-integration/rabbitmq),
and a forthcoming [version for Kubernetes](https://content.pivotal.io/blog/introducing-rabbitmq-for-kubernetes).
These distributions include all of the features of the open source
version, with some additional management features. Support agreements
are part of the commercial licensing.
<img src="/img/commercial/support-and-hosting-phone.svg" id='support-and-hosting-phone' />
## Support + Hosting
Pivotal Software provides [support for open source
RabbitMQ](https://pivotal.io/rabbitmq), available for a subscription
fee. The following companies provide technical support and/or cloud
hosting of open source RabbitMQ:
[CloudAMQP](https://www.cloudamqp.com/),
[Erlang Solutions](https://www.erlang-solutions.com/products/rabbitmq.html),
[AceMQ](https://acemq.com/rabbitmq/),
[Visual Integrator, Inc](http://www.visualintegrator.com/rmq/) and
[Google Cloud Platform](https://console.cloud.google.com/launcher/details/click-to-deploy-images/rabbitmq).
RabbitMQ can also be deployed in AWS and Microsoft Azure.
<img src="/img/commercial/testing-phone.svg" id='testing-phone' />
## Training
The following companies provide free, virtual, or instructor-led courses
for RabbitMQ:
[Pivotal Software](https://academy.pivotal.io/store-catalog),
[Erlang Solutions](https://www.erlang-solutions.com/products/rabbitmq.html),
[Visual Integrator, Inc](http://www.visualintegrator.com/rmq/) and
[LearnQuest](https://www.learnquest.com/course-detail-v3.aspx?cnum=rabbitmq-e1xc).
`}
</Md>
<div id=''>
</div>
</div>
</div>
);
}
}
class Community extends React.Component {
render() {
return (
<div id='community' className='home-section-container'>
<div className='home-section'>
<div className='tiles'>
<Md className='tile'>
{`
# Community
<a class="btn btn-primary" href="https://groups.google.com/forum/#!forum/rabbitmq-users">Mailing List</a>
<a class="btn btn-secondary" href="https://rabbitmq-slack.herokuapp.com/">Slack Channel</a>
Meet your fellow Rabbits to share stories, advice, and get help.
## Issues & Bug Reports
Start by searching the [Mailing
List](https://groups.google.com/forum/#!forum/rabbitmq-users) archive
and known issues on [Github](https://github.com/rabbitmq?q=rabbitmq).
It’s very likely fellow users have raised the same issue.
## Contributions
RabbitMQ welcomes contributions from the community. Please see our
[Contributors Page](/github.html) to learn more.
`}
</Md>
<Md className='tile'>
{`
# Contact Us
## Commercial inquiries
[Pivotal Sales](mailto:<EMAIL>) |
[Pivotal Support](https://support.pivotal.io)
## Other inquiries
[Contact us](/contact.html)
## Report a security vulnerability
[<EMAIL>](mailto:<EMAIL>)
## Social media
[YouTube](https://www.youtube.com/channel/UCSg9GRMGAo7euj3baJi4dOg) |
[Twitter](https://twitter.com/RabbitMQ)
`}
</Md>
</div>
</div>
</div>
);
}
}
class Index extends React.Component {
render() {
const {config: config, language = ''} = this.props;
return (
<div id="homepage">
<HomeHero config={config} language={language} />
<Intro config={config} language={language} />
<Features config={config} language={language} />
<GetStarted config={config} language={language} />
<Support config={config} language={language} />
<Community config={config} language={language} />
</div>
);
}
}
module.exports = Index;
<file_sep>---
id: index
title: Introduction
---
TODO<file_sep>---
id: use-management-ui
title: Use the management web UI
---
In addition to the CLI we discovered in the previous step, RabbitMQ
provides a web UI to learn about a particular node's configuration, load
and health.
> **TODO**: Write this page.
<file_sep>---
id: index
title: Messaging the RabbitMQ Way
---
In this guide we'll look at the various components of AMQP 0-9-1 such as exchanges, bindings, queues and how they are combined to produce different messaging patterns.
The simplest thing to remember is that:
1. Publishers open long lived connections to a RabbitMQ broker and send messages down a channel.
2. The channel on the broker side routes messages to queues based on routing rules determined by the exchange, exchange to queue bindings and message properties.
3. Messages are stored in queues in FIFO order and remain there until they can be delivered to a consumer.
4. Consumers subscribe to queues over long lived connections and get delivered messages in FIFO order.
## Logical Routing Architecture

## Physical Routing Architecture
In reality, exchanges are not things but simply meta-data that determine message routing. The real flow is publisher channel to queue to consumer channel.

Understanding the physical message flow can help you as you learn more about RabbitMQ clustering and performance.
Most diagrams will use the logical routing style to simplify things.<file_sep>---
id: publish-message
title: Publish a message
---
Now that RabbitMQ is running, we will publish our first message.
RabbitMQ supports several protocols to publish and consume messages and
client libraries are available for dozen of programming languages and
environments. For this tutorial, we will be using a Python AMQP 0-9-1
client library.
> **TODO**: Write this page.
<!--DOCUSAURUS_CODE_TABS-->
<!--Python-->
```python
#!/usr/bin/env python
# This client uses the Pika, an AMQP 0-9-1 client library.
import pika
# Open a connection, then a channel on that connection.
connection = pika.BlockingConnection(
pika.ConnectionParameters(host='localhost'))
channel = connection.channel()
# Declare a queue named "hello".
channel.queue_declare(queue='hello')
# Publish a message using the channel previously opened.
channel.basic_publish(exchange='', routing_key='hello', body='Hello World!')
print(" [x] Sent 'Hello World!'")
# Close the connection, which implicitly closes the channel.
connection.close()
```
<!--Java-->
```java
import com.rabbitmq.client.Channel;
import com.rabbitmq.client.Connection;
import com.rabbitmq.client.ConnectionFactory;
import java.nio.charset.StandardCharsets;
public class Send {
private final static String QUEUE_NAME = "hello";
public static void main(String[] argv) throws Exception {
ConnectionFactory factory = new ConnectionFactory();
factory.setHost("localhost");
/* Open a connection, then a channel on that connection. */
try (Connection connection = factory.newConnection();
Channel channel = connection.createChannel()) {
/* Declare a queue named "hello". */
channel.queueDeclare(QUEUE_NAME, false, false, false, null);
/* Publish a message using the channel previously opened. */
String message = "Hello World!";
channel.basicPublish(
"", QUEUE_NAME, null,
message.getBytes(StandardCharsets.UTF_8));
System.out.println(" [x] Sent '" + message + "'");
}
/* The connection is closed, as well as the channel, when they
* go out of scope. */
}
}
```
<!--.NET-->
```csharp
using System;
using RabbitMQ.Client;
using System.Text;
class Send
{
public static void Main()
{
var factory = new ConnectionFactory() { HostName = "localhost" };
using(var connection = factory.CreateConnection())
using(var channel = connection.CreateModel())
{
channel.QueueDeclare(
queue: "hello",
durable: false,
exclusive: false,
autoDelete: false,
arguments: null);
string message = "Hello World!";
var body = Encoding.UTF8.GetBytes(message);
channel.BasicPublish(
exchange: "",
routingKey: "hello",
basicProperties: null,
body: body);
Console.WriteLine(" [x] Sent {0}", message);
}
Console.WriteLine(" Press [enter] to exit.");
Console.ReadLine();
}
}
```
<!--END_DOCUSAURUS_CODE_TABS-->
<file_sep>---
id: index
title: Introduction
---
Reliability Guide
- Intro
- Characteristics of Robust Systems
- Safety In-Flight
- Safety At-Rest
- Avoiding Overload
- Understanding Flow Control
- Outbox pattern<file_sep>---
id: safety-vs-performance
title: Safety vs Performance
---
TODO<file_sep>---
id: opensuse
title: Install on OpenSUSE and SLES
sidebar_label: OpenSUSE
---
> **TODO**: Write this page.
<file_sep>---
id: federation
title: Federation
---
TODO<file_sep>---
id: pub-sub-others
title: Publish-Subscribe with the Topic, Direct and Header exchanges
sidebar_label: Pub-Sub with Other Exchanges
---
TODO<file_sep>---
id: ephemeral-infrastructure
title: Ephemeral Exchanges and Queues
---
TODO<file_sep>---
id: high-availability
title: High Availability
---
High availability is the ability of a system to handle failures and remain available/functioning. RabbitMQ must be run as a cluster with the use of replicated queues to achieve true high availability.
## Replicated Queues
RabbitMQ has two types of replicated queue:
- Quorum queues
- Classic HA queues
Today we recommend the usage of Quorum queues as they have stronger durability and availability than Classic HA queues.
Quorum queues use the battle-tested Raft protocol for message replication across multiple brokers. In a three node cluster, a single broker can be lost without message loss or unavailability. In a five node cluster up to two brokers can be lost.
## Redundant storage + Automated Deployment
Redundant storage offers data safety but not high availability by itself. However, in the event of a broker loss it would be possible to deploy a new broker and attach the storage device. When fully automated this could result in only minutes of downtime once the incident has been detected.<file_sep>---
id: hub-and-spoke
title: Hub and Spoke Topologies
---
TODO<file_sep>---
id: communication-patterns
title: Communication Patterns
---
## Communication Patterns Overview
There are many communications styles to choose from and RabbitMQ supports them all. We'll be teasing apart the following definitions and the impact they have on software architectures:
- Synchronous vs Asynchronous
- RPC vs Durable Messaging
- Point-to-point vs publish-subscribe
> NOTE: Synchronous / asynchronous communication != language threading model (like async/await, futures etc).
>
> They are separate concepts! Read on to understand why.
## Synchronous vs Asynchronous Communication

### Synchronous
Communication is **synchronous** when Service A makes a request to Service B and waits for a response. Service A might be using async/await or a future so it doesn't block, but that is an internal implementation detail, the fact is that one application is waiting for a response to its request to another service.
HTTP example:
1. Service A makes a call to the HTTP API of Service B.
2. Service A waits for a response.
3. Service B processes the request and sends an HTTP response to Service A.
4. Service A resumes execution of that thread/continuation.
Messaging example:
1. Service A sends Service B a message.
2. Service A waits for a response message in a reply queue.
3. Service B processes the request and sends a response to the indicated reply queue.
4. Service A consumes the message and resumes execution of that thread/continuation.
Synchronous communications require both services A and B to up and available. Uptime of a service is directly corelated to the uptime of its downstream synchronous communication dependencies.
### Asynchronous
Communication is **asynchronous** when either:
- Service A makes a request to Service B but does not wait around for a response. A response may come back at some indeterminate point in the future and even via a different communications channel.
- Service A makes a request to Service B and does not expect a response at all - fire-and-forget.
HTTP example:
1. Service A makes an HTTP POST call to Service B to process an order. Service B responds immediately with an HTTP 200, confirming it has received the request (but not processed it yet).
2. Service A continues to serve other requests.
2. Service B processes the order.
4. Service B makes call to Service A to confirm the order has been processed.
5. Service A executes some logic, such an notifying the customer that the order has been processed.
> Note that in this example, in a scaled out service, the instance of Service A that made the request may not be the one that processes the response.
Messaging example:
1. Service A sends a ProcessOrder message to the messaging system. Messaging system confirms receipt.
2. Service A continues to serve other requests.
3. Messaging system delivers the message to Service B.
4. Service B processes the order.
5. Service B sends an OrderProcessed to the messaging system. Messaging system confirms receipt.
6. Messaging system delivers the message to Service A.
7. Service A executes some logic, such an notifying the customer that the order has been processed.
> Note that for Service A to work, it does not need Service B to be up. Meanwhile Service B does not need Service A to be up in order to process the order and notify Service A that it has done so. Uptime now is now only coupled to the reliability of the messaging system itself.
## RPC vs Durable Messaging
### Remote Procedure Call (RPC)
RPC is like calling a function, only the call is made over the network. Examples of RPC are:
- HTTP
- TCP
RPC is a synchronous communications style and therefore requires both endpoints to be up. This style of programming is most comfortable for programmers as it mimicks the normal way that a programmer writes code. It also has excellent support in modern programming languages and frameworks with support for multiple data serialization formats:
- JSON
- XML
- Binary serialization like Protocol Buffers, Thrift
RPC requires Service A to know the address of Service B so it can make a call to it. This problem is either solved by a classic configuration system or by a service discovery mechanism such as Consul or service names in Kubernetes.
RPC calls can combine into a wide and/or deep call graph which can lead to some downsides.

The major downside of RPC is coupling which affects:
- uptime
- failure rate
- latency
**Service uptime** of Service A is tied to the uptime of all its dependencies.
- Service A -> B, C, D, E all with 95% uptime = 77% uptime for Service A
- Service A -> B, C, D, E all with 99% uptime = 95% uptime for Service A
**Failure rates** of an operation in Service A that requires 5 synchronous calls is multiplied by the failure rates of each downstream operation.
**Latency spikes** are more likely as we increase the number of synchronous calls to downstream dependencies. If all services have the following latency profile: p50=5ms, p99.9=100ms, p99.999=5s
Dependencies | Latency | Every X Operations
--|--|--
1 | 5s | 10000
1 | 100ms | 1000
5 | 5s | 2000
5 | 100ms | 200
10 | 5s | 1000
10 | 100ms | 100
20 | 5s | 500
20 | 100ms | 50
Tail latencies that individually are rare events become more common place as our call graph grows.
Many of these drawbacks may be mitigated and bounded given enough infrastructure, expertise and operational excellence, but at some dollar cost.
### Durable Messaging
Moving to an asynchronous model can avoid many of the issues caused by too heavy a reliance on a synchronous communications architecture.
A messaging system naturally supports asynchronous communication but adds additional benefits:
- Unavailability of downstream services does not necessarily affect the availability of Service A.
- Latency spikes in downstream services does not affect the latency profile of Service A.
- Processing failures don't necessarily affect the operations of Service A.
- The messaging system can absorb traffic spikes that might have caused downstream systems to crash under the load.
It also brings its own challenges and downsides.
The programming model may be less straight forward as the asynchronous style can require more indirection. Rather than waiting for the order to be processed by Service B, Service A may need to let the caller know the order has been received and notify later when the OrderProcessed event is received.
An operation that is now comprised of multiple independent asynchronous operations can be more hard to troubleshoot/detect when things go wrong and more difficult to resolve. But there are tools available such as:
- deadletter queues
- poisonous message queues
- message retries
### A mix of both
In all likelihood we shouldn't try to force the usage of only synchronous RPC or asynchronous message passing in our architecture but use a blend of both.

Authentication is an example of what naturally fits RPC. Sending an email notification is an example of what naturally fits an asynchronous operation. There may be a large gray area in the middle where both are candidates, each with their pros and cons.
## Point-to-point vs Publish-Subscribe
### Point-to-point
Point-to-point communications can be performed in either a synchronous or asynchronous style. What makes point-to-point is that as a single operation there is one service talking to just one other service. Service A knows it wants to talk to Service B and makes a request to it, either directly via a protocol such as HTTP or via messaging system that supports it.
The downsides of point-to-point are the extra coupling it brings, especially if we have multiple services which need to be communicated with to perform an action. For example, to create an order in Service A today we make the following point-to-point communications:
1. Notify Inventory service
2. Notify shipping service
3. Call notification service to send a confirmation email
Tomorrow we add communications to the Customer Care service, the Invoice service, the Marketing Campaign service and each time it means changing the code in Service A.
The usual reasons for point-to-point communications are:
- RPC
- Commands (a service tells another service to do something)
### Publish-Subscribe
Publish-Subscribe is an asynchronous style where the publisher of a message does not know (or generally care) who will consume the message. A single message could be delivered to a single service, 10, 1000 or no services at all. The point is that the publisher simply sends a message and forgets about it. Independently any number of services can subscribe to the messages they care about.
Service A publishes the OrderCreated event, and the following services subscribe to that event:
- Notifications service (so that it can send a confirmation email)
- Inventory service (so that it can update its stock)
- Shipping service (so that is can begin an internal workflow to prepare the order)
When Service A publishes an OrderCreated event, that event automaticvally gets forwarded to all three services.
Tomorrow when we add three new subscribers, it does not require any code changes to Service A.

Publish-subscribe is most often used to broadcast events. An event is simply a fact about something that has happened in the system. Events can cause a chain reaction of activity where more events are emitted and reacted to.<file_sep>---
id: consumers
title: Consumers
---
Consumers subscribe to a queue using either auto-acknowledgement or manual acknowledgement mode and also optionally define a prefetch value.
## Acknowledgements and Prefetch
Once a channel has been established between a broker and a consumer, the consumer subscribes to a queue. The channel process on the broker will then start pushing messages from the queue down the channel to the consumer.
Because RabbitMQ uses a push model, it needs a back-pressure mechanism to control the flow of messages, to prevent overpowering the consumer. That mechanism is the combination of message acknowledgements and prefetch.
The prefetch is the number of unacknowledged messages the channel will allow at any one time. For example, with a prefetch of 5:
- broker pushes 1, 2, 3, 4, 5
- consumer acknowledges 1
- broker pushes 6
- consumer acknowledges 2, 3, 4
- broker pushes 7, 8, 9
At all times, there are no more than 5 unacknowledged messages on the channel. Higher prefetches yield higher throughputs but also have downsides, see the TODO page for a deeper dive.
A consumer can configure auto-acknowledgement mode which means a message will be automatically acknowledged upon sending the message. This is an unsafe setting that can result in message loss, but provides higher throughput. It is not generally recommended.
## Competing Consumers
We can scale out consumption of a queue if a single consumer cannot handle the load by itself. We simply add more consumers to the queue in question and they will all get a portion of messages delivered to them.
We call multiple consumers on a single queue **competing consumers** because each consumer competes to consume the same set of messages. With three consumers subscribed to a single queue, each should get delivered about 1/3 of the messages.

## Non-competing Consumers
When you want three consumers to each process every single message of an exchange, then you need three queues. You tend to need this when you have multiple different services all needing to consume the same messages.

## Scaling Consumers Independently
One of RabbitMQ's super powers is the way that it decouples both publishers from consumers, but also different consumers from each other.
In other messaging systems that are based on topics, you must scale out the topic itself according to the needs of the slowest consumer. With RabbitMQ each subscribing service gets its own queue and can add as many consumers as it desires, independently of other subscribed services.
<file_sep>// vim:sw=2:et:
/**
* Copyright (c) 2020-present, Pivotal Software, Inc.
*
* This source code is licensed under the MIT license found in the
* LICENSE file in the root directory of this source tree.
*/
const React = require('react');
import { readFileSync } from 'fs';
const renderMarkdown = require('../node_modules/docusaurus/lib/core/renderMarkdown.js');
class Md extends React.Component {
content() {
if (this.props.file) {
var data = readFileSync(this.props.file, 'utf8');
return renderMarkdown(data);
} else {
return React.Children.map(this.props.children, child => {
if (typeof child === 'string') {
return renderMarkdown(child);
}
return child;
});
}
}
render() {
const Container = this.props.container;
if (this.props.id && this.props.className) {
return <Container
id={this.props.id}
className={this.props.className}
dangerouslySetInnerHTML={{__html: this.content()}} />;
} else if (this.props.id) {
return <Container
id={this.props.id}
dangerouslySetInnerHTML={{__html: this.content()}} />;
} else if (this.props.className) {
return <Container
className={this.props.className}
dangerouslySetInnerHTML={{__html: this.content()}} />;
} else {
return <Container
dangerouslySetInnerHTML={{__html: this.content()}} />;
}
}
}
Md.defaultProps = {
container: 'div',
};
module.exports = Md;
<file_sep>---
id: index
title: Operations Guides
---
- Reference
- Installation
- All Packages and Repositories
- Debian and Ubuntu
- RHEL, CentOS, Fedora
- Windows
- Generic UNIX Binary Build
- MacOS via Standalone Binary Build
- MacOS via Homebrew
- Amazon EC2
- Solaris
- Configuration Management Tools
- Docker
- Kubernetes
- Package Signatures
- Supported Erlang/OTP Versions
- Supported RabbitMQ Versions
- Changelog
- Snapshot (Nightly) Builds
- Upgrades and OS Patching
- Full Cluster Upgrade/Restart
- Rolling Upgrades/Restarts
- Rolling Back?
- Configuration
- Reference
- File and Directory Locations
- Environment Variables
- Runtime Parameters
- CLI Tools
- Overview
- rabbitmqctl
- rabbitmqadmin
- rabbitmqqueues
- Multi DC and Multi-Cluster
- Intro
- Federation
- Shovel
- Securing Multi-Cluster Communications
- Monitoring/Observability
- Management UI and HTTP API
- Logging
- Metrics
- Health Checks
- Prometheus Support
- InfluxDB Support
- Grafana
- Troubleshooting guidance
- Client Connections
- AMQP 0-9-1 Channels
- Internal Event Exchange
- Per Virtual Host Limits
- Message Tracing
- Capturing Traffic with Wireshark
- Security
- Authentication and Authorisation
- Authentication and authorisation Backends
- Authentication Mechanisms
- Virtual Hosts
- Credentials and Passwords
- LDAP
- x509 Certificate-based Authentication
- Validated User ID
- Authentication Failure Notifications
- Networking and TLS
- Client Connections
- Networking
- Troubleshooting Network Connectivity
- Using TLS for Client Connections
- Using TLS for Inter-node Traffic
- Troubleshooting TLS
- Guides
- Production and Day Two Guides
- Production Checklist
- Monitoring with Prometheus and Grafana
- Monitoring with Elastic
- Disaster Recovery Strategies
- Troubleshooting Guide
- Reliability Guide
- Intro
- Safety At-Rest
- Avoiding Overload
- Understanding Flow Control
- Multi-DC Failovers??
- Performance Guide
- Intro
- Queue Types and Performance
- CPU
- Memory
- Disk
- Network
- Docker
- Upgrade Guide
- Intro
- High Availability Upgrade Strategies
- Rolling Upgrades
- Blue/Green Deployment Upgrades
- Cluster Expansion Upgrades
- Data Safe Upgrade Strategies
- Full Cluster Upgrade
- Blue/Green Deployment Upgrades
<file_sep>---
id: index
title: Welcome to the Developer Guide
sidebar_label: Introduction
---
See our [guides and tutorials](guides/index) that will help you learn how to combine various RabbitMQ features to achieve your messaging architecture objectives.
See our [developer reference](reference/index) documentation to learn the details about specific features.<file_sep>---
id: index
title: How to get RabbitMQ
---
## Trying RabbitMQ <span class="h-addition">(without installing)</span>
You can play with RabbitMQ to help you decide if it is the right tool
for you.
**The easiest to try RabbitMQ:**
```sh
curl -sSf https://rabbitmq.netlify.com/try-rabbitmq.sh | sh
```
For more detailed instructions to try RabbitMQ, here are two approaches.
The script above implements the first one.
<div class="doc-tiles"></div>
* [](precompiled)
### [Precompiled binaries](precompiled)
For both Unix systems and Microsoft Windows
* [](docker)
### [Docker](docker)
Those methods are also useful when you want to run RabbitMQ as an
unprivileged user.
## Installing RabbitMQ
<div class="doc-tiles"></div>
* [](debian)
### [Debian](debian)
Debian, Ubuntu and derivatives
* [](redhat)
### [Red Hat](redhat)
RHEL, CentOS, Fedora and derivatives
* [](opensuse)
### [OpenSUSE](opensuse)
OpenSUSE, SLES and derivatives
* [](windows)
### [Microsoft Windows](windows)
* [](freebsd)
## [FreeBSD](freebsd)
FreeBSD and derivatives
* [](macos)
## [macOS](macos)
## Build from source
If you want to work on RabbitMQ or if you are a package maintainer, you
can [build from source](build), either using a release source archive or
from a Git clone.
<file_sep>---
id: flow-control
title: Understanding Flow Control
---
TODO<file_sep>---
id: peer-clusters
title: Peer Clusters
---
TODO<file_sep>---
id: use-mqtt
title: Use MQTT
---
So far, we only seen how to publish and consume messages using AMQP
0-9-1. However, RabbitMQ supports many more protocols! MQTT is one of
them, let's see how to use it with RabbitMQ.
> **TODO**: Write this page.
<file_sep>---
id: configure-rabbitmq
title: Configure RabbitMQ
---
If you reached this tutorial:
* you know how to develop a client to publish and consume messages
* you know how to observe your RabbitMQ node.
Now, RabbitMQ offers many settings you may want to tweak to adapt it to
your workload. Let's see how this is done with two simple settings.
> **TODO**: Write this page.
<file_sep>---
id: priority-routing
title: Priority Based Routing / Class of Service
sidebar_label: Priority Based Routing
---
TODO<file_sep>---
id: index
title: Introduction
---
Intro to these guides...
TODO<file_sep>---
id: index
title: Getting Started
---
In this Getting Started guide, we will go through several steps to give
you a general idea of all the features of RabbitMQ. You will learn:
* Start RabbitMQ.
* How to configure your node.
* How to publish and consume messages, using different protocols.
* How to cluster many nodes to get high availibility.
* How to observe your RabbitMQ node.
<div class="getting-started-certificate"></div>

<file_sep>---
id: index
title: Federation and Shovel
---
TODO<file_sep>---
id: index
title: Virtual Hosts
---
TODO<file_sep>---
id: messages
title: Messages
---
## Message Properties
### AMQP 0-9-1
Every delivery combines message metadata and delivery information. Different client libraries use slightly different ways of providing access to those properties. Typically delivery handlers have access to a delivery data structure.
The following properties are delivery and routing details; they are not message properties per se and set by RabbitMQ at routing and delivery time:
| Property | Type | Description |
| -- | -- | -- |
| Delivery tag | Positive integer | Delivery identifier, see Confirms.|
| Redelivered | Boolean | Set to `true` if this message was previously delivered and requeued |
| Exchange | String | Exchange which routed this message |
| Routing key | String | Routing key used by the publisher |
| Consumer tag | String | Consumer (subscription) identifier |
The following are message properties. Most of them are optional. They are set by publishers at the time of publishing:
| Property | Type | Description | Required? |
| -- | -- | -- | -- |
| Delivery mode | Enum (1 or 2) | 2 for "persistent", 1 for "transient". Some client libraries expose this property as a boolean or enum. | Yes |
| Type | String | Application-specific message type, e.g. "orders.created" | No |
| Headers | Map (string => any) | An arbitrary map of headers with string header names | No |
| Content type | String | Content type, e.g. "application/json". Used by applications, not core RabbitMQ | No |
| Content encoding | String | Content encoding, e.g. "gzip". Used by applications, not core RabbitMQ | No |
| Message ID | String | Arbitrary message ID | No |
| Correlation ID | String | Helps correlate requests with responses, see tutorial 6 | No |
| Reply To | String | Carries response queue name, see tutorial 6 | No |
| Expiration | String | Per-message TTL | No |
| Timestamp | Timestamp | Application-provided timestamp | No |
| User ID | String | User ID, validated if set | No |
| App ID | String | Application name | No |
## Message Types
The type property on messages is an arbitrary string that helps applications communicate what kind of message that is. It is set by the publishers at the time of publishing. The value can be any domain-specific string that publishers and consumers agree on.
RabbitMQ does not validate or use this field, it exists for applications and plugins to use and interpret.
Message types in practice naturally fall into groups, a dot-separated naming convention is common (but not required by RabbitMQ or clients), e.g. orders.created or logs.line or profiles.image.changed.
If a consumer gets a delivery of an unknown type it is highly advised to log such events to make troubleshooting easier.
## Content Type and Encoding
The content (MIME media) type and content encoding fields allow publishers communicate how message payload should be deserialized and decoded by consumers.
RabbitMQ does not validate or use these fields, it exists for applications and plugins to use and interpret.
For example, messages with JSON payload should use application/json. If the payload is compressed with the LZ77 (GZip) algorithm, its content encoding should be gzip.
Multiple encodings can be specified by separating them with commas.<file_sep>---
id: protocols
title: Protocols
---
RabbitMQ natively supports AMQP 0.9.1 but also can be configured to support extra protocols:
- MQTT
- STOMP
- AMQP 1.0
## MQTT
MQTT is a publish-subscribe messaging protocol used commonly in IOT.
- Clients can publish messages to topics on a broker.
- Clients can subscribe to individual topics or a hierarchy of topics.
- Supports three levels of delivery guarantee (QoS) scoped to publisher-broker and broker-consumer:
- QoS 0: At-Most-Once delivery (messages can be lost)
- QoS 1: At-Least-Once delivery (messages cannot be lost, but can be delivered more than once)
- QoS 2: Exactly-Once (messages are delivered exactly once)
Exactly-Once only makes guarantees about delivery from publisher to broker, and broker to consumer. It does not prevent:
- a broker from losing the message. It is up to the broker to implement message replication or the server administrator to provide redundant storage.
- a consumer using a different QoS less strong than Exactly-Once.
RabbitMQ supports all MQTT 3.1 features except for QoS 2 for consumers. It does however provide the ability to use replicated queues providing high availability and data safety.
- Link to plugin in docs TODO
- [GitHub](https://github.com/rabbitmq/rabbitmq-mqtt)
## STOMP
STOMP stands for Simple (or Streaming) Text Orientated Messaging Protocol. It is a text based publish-subscribe messaging protocol that is designed to be simple and lightweight.
Clients (both publishers and subscribers) open long lived connections to a broker. Messages are published to **destinations** and consumers subscribe to **destinations**. It does not include any message durability and persistence features in the protocol itself and the RabbitMQ implementation relies on the usual durability guarantees you get with any queue.
RabbitMQ offers many different ways to map STOMP destinations onto RabbitMQ exchanges and queues. Two plugins are provided.
### STOMP plugin
Read more about the STOMP plugin:
- Link to plugin in docs TODO
- [GitHub](https://github.com/rabbitmq/rabbitmq-stomp)
### Web STOMP plugin (STOMP over WebSocket)
Provides for support for STOMP over WebSocket to allow for browser to broker communications.
Read more about the Web STOMP plugin:
- Link to web plugin in docs TODO
- [GitHub](https://github.com/rabbitmq/rabbitmq-web-stomp)
## AMQP 1.0
AMQP 1.0 is a completely different protocol to AMQP 0.9.1 and should be considered separate protocols. AMQP 1.0 has wide support in various messaging systems, including RabbitMQ. However, RabbitMQ supports only a subset of the full protocol.
This [YouTube this video series from Microsoft](https://www.youtube.com/watch?v=ODpeIdUdClc) as a good AMQP 1.0 primer.
Learn more about RabbitMQ's support for AMQP 1.0:
- [GitHub](https://github.com/rabbitmq/rabbitmq-amqp1.0)
- Developer guide page TODO
Read on to learn about the differences between AMQP 0.9.1 and AMQP 1.0.
### Peer-to-peer (1.0) vs Broker Based (0.9.1)
AMQP 1.0 is a peer-to-peer protocol, it does not include the concept of a broker. Therefore it does not natively include routing, publish-subscribe or message durability. Despite not being a part of the protocol, typically AMQP 1.0 is used in a broker based system where one endpoint is a broker and the other an application. Through broker implementations you typically get publish-subscribe features.
AMQP 0.9.1 is an overtly broker-based protocol with native support for routing and publish-subscribe.
### Connection Concepts and Flow Control
AMQP 1.0 has a more complex set of concepts including connections, channels, sessions, nodes and links. It has two levels of flow control (session level and link level) to prevent system resources being used up and to throttle fast publishers.
AMQP 0.9.1 has a model of connections, channels, exchanges and queues. Flow control exists at three levels: the connection, channel and queue level. Addtionally, consumers can perform flow control by the use of prefetch and acknowledgements.
### On the wire format
AMQP 1.0 has a more complex on the wire binary format more similar to something like Avro, ProtoBuf or Thrift. AMQP 0.9.1 simply stores bytes as the publisher provides.<file_sep>---
id: reference
title: Policies Reference
---
TODO
| 483b9931c55461b0860fa81b0d9b0ee964cd7d39 | [
"Markdown",
"JavaScript",
"Shell"
] | 107 | Markdown | isabella232/testing-docusaurus | 9acf9f26a5b1421552aac55cff4977fc03f9002f | 8fdee37ab6f35f58b2b58bf65ad852a9ac66ab10 | |
refs/heads/master | <file_sep>
const task = {
title: 'Buy Milk',
description: 'go to shop and buy a milk'
};
Object.defineProperty(task, 'toString', {
value: function() {
return this.title + ' ' + this.description;
},
writable: false,
configurable: false,
enumerable: false // if omitted or set to false won't be displayed or iterated over
});
const urgentTask = Object.create(task);
Object.defineProperty(urgentTask, 'toString', {
value: function() {
return this.title + ' is urgent';
},
writable: false,
configurable: false,
enumerable: false
});
console.log(urgentTask.toString()); // Buy Milk is urgent<file_sep>
const Task = require('./task');
const Repo = require('./taskRepository');
let task = new Task(Repo.get(1));
let task1 = new Task({ name: 'Run' });
let task2 = new Task({ name: 'Shop' });
let task3 = new Task({ name: 'Play' });
task.complete();
task1.complete();
task2.save();
task3.save();
<file_sep>
const Task = require('./task');
const taskRepo = require('./taskRepo');
const userRepo = require('./userRepo');
const projectRepo = require('./projectRepo');
let task = new Task(taskRepo.get(1));
const user = userRepo.get(1);
const project = projectRepo.get(1);
task.user = user;
task.project = project;
console.log(task);
task.save();
<file_sep>
const Task = require('./task');
const repoFactory = require('./repoFactory');
let task = new Task(repoFactory.getRepo('task').get(1));
const user = repoFactory.getRepo('user').get(1);
const project = repoFactory.getRepo('project').get(1);
task.user = user;
task.project = project;
console.log(task);
task.save();
<file_sep>
const Task = require('./task');
const repoFactory = require('./repoFactory2');
let task = new Task(repoFactory.task.get(1));
const user = repoFactory.user.get(1);
const project = repoFactory.project.get(1);
task.user = user;
task.project = project;
console.log(task);
task.save();
<file_sep>
## Practical Design Patterns in JavaScript
Author: **<NAME>**
Practiced: **36/57**
Watched: **48/57**
| 397f9056468468aec3c4794a3c2f71f83fcb1123 | [
"JavaScript",
"Markdown"
] | 6 | JavaScript | stepanenko/john-mills-design-patterns | 61131aa4e465f6800b29df4c3f767ef7c0f59635 | e6998281704cd458446c70b7f2bb9e85ab6930f1 | |
refs/heads/master | <repo_name>L2-Zhang/Bayesian-Optimization<file_sep>/bo.py
# -*- coding: utf-8 -*-
"""
Created on Fri Sep 18 18:41:19 2020
@author: zhangliangliang
"""
import numpy as np
import matplotlib.pyplot as plt
from scipy.special import gamma, kv
from scipy.spatial.distance import cdist
from collections import OrderedDict
import numpy as np
from joblib import Parallel, delayed
from scipy.optimize import minimize
from scipy.stats import norm, t
class bcolors:
HEADER = '\033[95m'
OKBLUE = '\033[94m'
OKGREEN = '\033[92m'
WARNING = '\033[93m'
FAIL = '\033[91m'
ENDC = '\033[0m'
BOLD = '\033[1m'
UNDERLINE = '\033[4m'
default_bounds = {
'l': [1e-4, 1],
'sigmaf': [1e-4, 2],
'sigman': [1e-6, 2],
'v': [1e-3, 10],
'gamma': [1e-3, 1.99],
'alpha': [1e-3, 1e4],
'period': [1e-3, 10]
}
class EventLogger:
def __init__(self, gpgo):
self.gpgo = gpgo
self.header = 'Evaluation \t Proposed point \t Current eval. \t Best eval.'
self.template = '{:6} \t {}. \t {:6} \t {:6}'
print(self.header)
def _printCurrent(self, gpgo):
eval = str(len(gpgo.GP.y) - gpgo.init_evals)
proposed = str(gpgo.best)
curr_eval = str(gpgo.GP.y[-1])
curr_best = str(gpgo.tau)
if float(curr_eval) >= float(curr_best):
curr_eval = bcolors.OKGREEN + curr_eval + bcolors.ENDC
print(self.template.format(eval, proposed, curr_eval, curr_best))
def _printInit(self, gpgo):
for init_eval in range(gpgo.init_evals):
print(self.template.format('init', gpgo.GP.X[init_eval], gpgo.GP.y[init_eval], gpgo.tau))
def l2norm_(X, Xstar):
return cdist(X, Xstar)
def kronDelta(X, Xstar):
return cdist(X, Xstar) < np.finfo(np.float32).eps
class matern52:
def __init__(self, l=1, sigmaf=1, sigman=1e-6, bounds=None, parameters=['l', 'sigmaf', 'sigman']):
self.l = l
self.sigmaf = sigmaf
self.sigman = sigman
self.parameters = parameters
if bounds is not None:
self.bounds = bounds
else:
self.bounds = []
for param in self.parameters:
self.bounds.append(default_bounds[param])
def K(self, X, Xstar):
r = l2norm_(X, Xstar)/self.l
one = (1 + np.sqrt(5 * r ** 2) + 5 * r ** 2 / 3)
two = np.exp(-np.sqrt(5 * r ** 2))
return self.sigmaf * one * two + self.sigman * kronDelta(X, Xstar) #如果cdist是一个极小值,就加上sigman
import numpy as np
from scipy.linalg import cholesky, solve
from collections import OrderedDict
from scipy.optimize import minimize
class GaussianProcess:
def __init__(self, covfunc, optimize=False, usegrads=False, mprior=0):
self.covfunc = covfunc
self.optimize = optimize
self.usegrads = usegrads
self.mprior = mprior
def getcovparams(self):
d = {}
for param in self.covfunc.parameters:
d[param] = self.covfunc.__dict__[param]
return d
def fit(self, X, y):
self.X = X
self.y = y
self.nsamples = self.X.shape[0]
if self.optimize:
grads = None
if self.usegrads: grads = self._grad
self.optHyp(param_key=self.covfunc.parameters, param_bounds=self.covfunc.bounds, grads=grads)
self.K = self.covfunc.K(self.X, self.X)
# print("self.K ",self.K )
# self.alpha = solve(self.K, y - self.mprior)
# print("self.alpha ",self.belta )
#it is typically faster and more numerically stable to use a Cholesky decomposition
self.L = cholesky(self.K).T
self.alpha = solve(self.L.T, solve(self.L, y - self.mprior))
# print("self.alpha",self.alpha)
# print("self.y",self.y)
# print("np.dot(self.y, self.alpha)",np.dot(self.y, self.alpha))
# self.logp = -.5 * np.dot(self.y, self.alpha) - np.sum(np.log(np.diag(self.L))) - self.nsamples / 2 * np.log( 2 * np.pi)
def param_grad(self, k_param):
k_param_key = list(k_param.keys())
covfunc = self.covfunc.__class__(**k_param)
K = covfunc.K(self.X, self.X)
L = cholesky(K).T
alpha = solve(L.T, solve(L, self.y))
inner = np.dot(np.atleast_2d(alpha).T, np.atleast_2d(alpha)) - np.linalg.inv(K)
grads = []
for param in k_param_key:
gradK = covfunc.gradK(self.X, self.X, param=param)
gradK = .5 * np.trace(np.dot(inner, gradK))
grads.append(gradK)
return np.array(grads)
def predict(self, Xstar, return_std=False):
Xstar = np.atleast_2d(Xstar)
kstar = self.covfunc.K(self.X, Xstar).T
fmean = self.mprior + np.dot(kstar, self.alpha)
v = solve(self.L, kstar.T)
fcov = self.covfunc.K(Xstar, Xstar) - np.dot(v.T, v)
if return_std:
fcov = np.diag(fcov)
return fmean, fcov
def update(self, xnew, ynew):
y = np.concatenate((self.y, ynew), axis=0)
X = np.concatenate((self.X, xnew), axis=0)
self.fit(X, y)
class Acquisition:
def __init__(self, mode, eps=1e-06, **params):
self.params = params
self.eps = eps
mode_dict = {
'ExpectedImprovement': self.ExpectedImprovement,
}
self.f = mode_dict[mode]
def ExpectedImprovement(self, tau, mean, std):
z = (mean - tau - self.eps) / (std + self.eps)
return (mean - tau) * norm.cdf(z) + std * norm.pdf(z)[0]
def eval(self, tau, mean, std):
return self.f(tau, mean, std, **self.params)
class GPGO:
def __init__(self, surrogate, acquisition, f, parameter_dict, n_jobs=1):
self.GP = surrogate
self.A = acquisition
self.f = f
self.parameters = parameter_dict
self.n_jobs = n_jobs
self.parameter_key = list(parameter_dict.keys())
self.parameter_value = list(parameter_dict.values())
self.parameter_type = [p[0] for p in self.parameter_value]
self.parameter_range = [p[1] for p in self.parameter_value]
self.history = []
self.logger = EventLogger(self)
def _sampleParam(self):
d = OrderedDict()
for index, param in enumerate(self.parameter_key):
if self.parameter_type[index] == 'int':
d[param] = np.random.randint(self.parameter_range[index][0], self.parameter_range[index][1])
elif self.parameter_type[index] == 'cont':
d[param] = np.random.uniform(self.parameter_range[index][0], self.parameter_range[index][1])
else:
raise ValueError('Unsupported variable type.')
return d
def _firstRun(self, n_eval=3):
self.X = np.empty((n_eval, len(self.parameter_key)))
self.y = np.empty((n_eval,))
for i in range(n_eval): #随机生成n_eval个点(xi,yi),并使用GP.fit()
s_param = self._sampleParam()
s_param_val = list(s_param.values())
self.X[i] = s_param_val
self.y[i] = self.f(**s_param)
self.GP.fit(self.X, self.y)
self.tau = np.max(self.y)
self.history.append(self.tau)
def _acqWrapper(self, xnew):
new_mean, new_var = self.GP.predict(xnew, return_std=True)
new_std = np.sqrt(new_var + 1e-6)
return -self.A.eval(self.tau, new_mean, new_std)
def _optimizeAcq(self, method='L-BFGS-B', n_start=100):
start_points_dict = [self._sampleParam() for i in range(n_start)]
start_points_arr = np.array([list(s.values()) for s in start_points_dict])
x_best = np.empty((n_start, len(self.parameter_key)))
f_best = np.empty((n_start,))
if self.n_jobs == 1:
for index, start_point in enumerate(start_points_arr):
res = minimize(self._acqWrapper, x0=start_point, method=method,bounds=self.parameter_range)
# print("res:",res.x, np.atleast_1d(res.fun)[0])
x_best[index], f_best[index] = res.x, np.atleast_1d(res.fun)[0]
else:
opt = Parallel(n_jobs=self.n_jobs)(delayed(minimize)(self._acqWrapper, x0=start_point,method=method,
bounds=self.parameter_range) for start_point in start_points_arr)
x_best = np.array([res.x for res in opt])
f_best = np.array([np.atleast_1d(res.fun)[0] for res in opt])
self.best = x_best[np.argmin(f_best)]
def updateGP(self):
kw = {param: self.best[i] for i, param in enumerate(self.parameter_key)}
f_new = self.f(**kw)
self.GP.update(np.atleast_2d(self.best), np.atleast_1d(f_new))
self.tau = np.max(self.GP.y)
self.history.append(self.tau)
def getResult(self):
argtau = np.argmax(self.GP.y)
opt_x = self.GP.X[argtau]
res_d = OrderedDict()
for i, (key, param_type) in enumerate(zip(self.parameter_key, self.parameter_type)):
if param_type == 'int':
res_d[key] = int(opt_x[i])
else:
res_d[key] = opt_x[i]
return res_d, self.tau
def run(self, max_iter=10, init_evals=3, resume=False):
if not resume:
self.init_evals = init_evals
self._firstRun(self.init_evals)
self.logger._printInit(self)
for iteration in range(max_iter):
self._optimizeAcq()
self.updateGP()
self.logger._printCurrent(self)
def drawFun(f):
x = np.linspace(0, 1, 1000)
plt.plot(x, f(x))
plt.grid()
plt.show()
def f(x):
return -((6*x-2)**2)
# return (x-2)**2
if __name__ == '__main__':
np.random.seed(20)
drawFun(f)
sexp = matern52()
gp = GaussianProcess(sexp)
acq = Acquisition(mode = 'ExpectedImprovement')
params = {'x': ('cont', (-2, 4))}
gpgo = GPGO(gp, acq, f, params)
gpgo.run(max_iter = 20)
print(gpgo.getResult())
<file_sep>/README.md
# Bayesian-Optimization
simple realization of Bayesian Optimization
Refer to https://github.com/josejimenezluna/pyGPGO/tree/master/pyGPGO.
Cov_func: matern52
Acquisition: ExpectedImprovement
| 24a197806e37b7e52efefedd502035fc194a95bc | [
"Markdown",
"Python"
] | 2 | Python | L2-Zhang/Bayesian-Optimization | 7659fcf99c5c34f2c958322a0a8b9c7082959e93 | 07e5b7b10a635c88202f7f0c6bf42676764d5d93 | |
refs/heads/development | <file_sep>import Link from "next/link";
import { allArticles } from ".contentlayer/generated";
import { pick } from "@contentlayer/client";
import Article from "components/article";
export default function Home({ articles }) {
return (
<div>
<main className="font-mono max-w-3xl mx-auto pt-10 pb-40">
<h1 className="text-3xl px-5"><NAME></h1>
<section className="pt-12">
<div className="w-full">
{articles.map((article) => (
<Article key={article.slug} article={article} />
))}
</div>
</section>
</main>
</div>
);
}
export async function getStaticProps() {
const articles = allArticles.map((article) => pick(article, ["title", "date", "readingTime", "slug", "thumbnail"]));
return { props: { articles } };
}
| 8fd82db0d77917861e7919efcacb12258ff009d6 | [
"JavaScript"
] | 1 | JavaScript | erikfloresq/erikfloresq.github.io | 8fa0ed5d6343ff36da89fddbb867a46924689bc4 | a84543cfe4bb7f5b52e2fdf34f7a2c80d171763a | |
refs/heads/master | <file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using CalcualtorApp.Models;
using Microsoft.AspNetCore.Mvc;
// For more information on enabling MVC for empty projects, visit https://go.microsoft.com/fwlink/?LinkID=397860
namespace CalcualtorApp.Controllers
{
public class CalculatorController : Controller
{
// GET: /<controller>/
public IActionResult Calculator()
{
return View();
}
public IActionResult Calculate(Calculator calculator)
{
if(calculator.Operator == '+')
{
calculator.Result = calculator.Number1 + calculator.Number2;
}
else if(calculator.Operator == '-')
{
calculator.Result = calculator.Number1 - calculator.Number2;
}
else if(calculator.Operator == '*')
{
calculator.Result = calculator.Number1 * calculator.Number2;
}
else if(calculator.Operator == '/')
{
calculator.Result = calculator.Number1 / calculator.Number2;
}
return View("Calculator", calculator);
}
}
}
| b3a1893ab0ac3ba12a35b150ef173a6420384f8a | [
"C#"
] | 1 | C# | LucasCarver/CalculatorWebApp | 12ad09b191e80542b95b7f6eb28486411cda0bca | 806bc545ec97648baf4daabae7dda4061195644c | |
refs/heads/master | <repo_name>HugoJF/minecraft-autoreplanter<file_sep>/src/main/java/com/denerdtv/AutoReplanterCommand.java
package com.denerdtv;
import org.bukkit.command.Command;
import org.bukkit.command.CommandExecutor;
import org.bukkit.command.CommandSender;
import org.bukkit.command.TabCompleter;
import org.bukkit.entity.Player;
import org.bukkit.event.Listener;
import java.util.ArrayList;
import java.util.List;
import static com.denerdtv.AutoReplanter.SERVER_PREFIX;
import static org.bukkit.ChatColor.*;
public class AutoReplanterCommand implements Listener, CommandExecutor, TabCompleter {
private final AutoReplanter ar;
public AutoReplanterCommand() {
this.ar = AutoReplanter.getInstance();
}
@Override
public boolean onCommand(CommandSender sender, Command cmd, String label, String[] args) {
if (cmd.getName().equalsIgnoreCase(AutoReplanter.COMMAND)) {
Player p = (Player) sender;
if (args.length == 1 && args[0].equals("on")) {
ar.setEnabled(p, true);
p.sendMessage(SERVER_PREFIX + "AutoReplanter is now turned " + GREEN + "ON");
return true;
} else if (args.length == 1 && args[0].equals("off")) {
ar.setEnabled(p, false);
p.sendMessage(SERVER_PREFIX + "AutoReplanter is now turned " + RED + "OFF");
return true;
} else if (args.length == 1 && args[0].equalsIgnoreCase("visible")) {
ar.setShowing(true);
p.sendMessage(SERVER_PREFIX + "AutoReplanter is now " + GREEN + "VISIBLE");
return true;
} else if (args.length == 1 && args[0].equalsIgnoreCase("hide")) {
ar.setShowing(false);
p.sendMessage(SERVER_PREFIX + "AutoReplanter is now " + RED + "HIDDEN");
return true;
} else if (args.length == 1 && args[0].equalsIgnoreCase("status")) {
p.sendMessage(SERVER_PREFIX + "Currently tracking " + ar.getLocationCount() + " farms");
return true;
} else if (args.length == 1 && args[0].equalsIgnoreCase("chests")) {
p.sendMessage(SERVER_PREFIX + "Currently working with " + AutoReplanter.getInstance().getChests().size() + " chests");
return true;
} else {
p.sendMessage(SERVER_PREFIX + ("Usage: AutoReplanter <" + RED + "on|off|visible|hide|status|chests" + RESET + ">").replaceAll("\\|", RESET + "|" + RED));
}
}
return false;
}
@Override
public List<String> onTabComplete(CommandSender sender, Command cmd, String label, String[] args) {
List<String> cmds = new ArrayList<>();
Player p = (Player) sender;
// Generate TAB complete for AutoReplant
if (cmd.getName().equalsIgnoreCase(AutoReplanter.COMMAND)) {
generateEnabledTabComplete(cmds, p);
generateVisibilityTabComplete(cmds);
generateStatusTabComplete(cmds);
generateChestsTabComplete(cmds);
}
// Filter entries based on already typed arguments
filterBasedOnHints(args, cmds);
return cmds;
}
private void generateChestsTabComplete(List<String> cmds) {
cmds.add("chests");
}
private void generateStatusTabComplete(List<String> cmds) {
cmds.add("status");
}
private void generateVisibilityTabComplete(List<String> cmds) {
if (!ar.getShowing()) cmds.add("visible");
if (ar.getShowing()) cmds.add("hide");
}
private void generateEnabledTabComplete(List<String> cmds, Player p) {
boolean off = ar.getEnabled(p);
if (!off) cmds.add("on");
if (off) cmds.add("off");
}
private void filterBasedOnHints(String[] args, List<String> cmds) {
if (args.length >= 1) {
for (int i = 0; i < cmds.size(); i++) {
if (!cmds.get(i).startsWith(args[0])) {
cmds.remove(i--);
}
}
}
}
}
| 1c349b27fba71e40fb591bf93875bb366588b99c | [
"Java"
] | 1 | Java | HugoJF/minecraft-autoreplanter | a9225dd2f9d3d7b17a0630f70cbefdec9f9b9a79 | bdf02bec4f6cd908190aa2d89c724ad18069d1cc | |
refs/heads/master | <file_sep>// tslint:disable-next-line:no-var-requires
const path = require('path')
process.env.TS_NODE_PROJECT = path.resolve('test/tsconfig.json')
<file_sep>import {expect, test} from '@oclif/test'
import * as chai from 'chai'
import {describe} from 'mocha'
import * as sinon from 'sinon'
import Snapshot from '../../src/commands/snapshot'
import { DEFAULT_CONFIGURATION } from '../../src/configuration/configuration'
import {AgentService} from '../../src/services/agent-service'
import StaticSnapshotService from '../../src/services/static-snapshot-service'
import {captureStdOut} from '../helpers/stdout'
describe('snapshot', () => {
describe('#run', () => {
const sandbox = sinon.createSandbox()
afterEach(() => {
sandbox.restore()
})
function AgentServiceStub(): AgentService {
const agentService = AgentService.prototype as AgentService
sandbox.stub(agentService, 'start')
const start = new Snapshot([], '') as Snapshot
sandbox.stub(start, 'agentService').returns(agentService)
return agentService
}
function StaticSnapshotServiceStub(): StaticSnapshotService {
const staticSnapshotService = StaticSnapshotService.prototype as StaticSnapshotService
sandbox.stub(staticSnapshotService, 'snapshotAll')
sandbox.stub(staticSnapshotService, 'start')
return staticSnapshotService
}
it('starts the static snapshot service', async () => {
const agentServiceStub = AgentServiceStub()
const staticSnapshotServiceStub = StaticSnapshotServiceStub()
const stdout = await captureStdOut(async () => {
await Snapshot.run(['.'])
})
chai.expect(agentServiceStub.start).to.be.calledWith(DEFAULT_CONFIGURATION)
chai.expect(staticSnapshotServiceStub.start).to.have.callCount(1)
chai.expect(staticSnapshotServiceStub.snapshotAll).to.have.callCount(1)
chai.expect(stdout).to.match(/\[percy\] percy has started./)
})
})
describe('snapshot command', () => {
test
.stub(process, 'env', {PERCY_TOKEN: ''})
.stderr()
.command(['snapshot', './test_dir'])
.exit(0)
.do((output) => expect(output.stderr).to.contain(
'Warning: Skipping visual tests. PERCY_TOKEN was not provided.',
))
.it('warns about PERCY_TOKEN not being set and exits gracefully')
test
.env({PERCY_TOKEN: 'abc'})
.command(['snapshot'])
.exit(2)
.it('exits when the asset directory arg is missing')
})
})
<file_sep>import * as std from 'stdout-stderr'
export async function captureStdOut(callback: any): Promise<string> {
std.stdout.start()
await callback()
std.stdout.stop()
return std.stdout.output
}
export async function captureStdErr(callback: any): Promise<string> {
std.stderr.start()
await callback()
std.stderr.stop()
return std.stderr.output
}
<file_sep>import * as chai from 'chai'
import {describe} from 'mocha'
import * as nock from 'nock'
import * as sinon from 'sinon'
import Exec from '../../src/commands/exec'
import {AgentService} from '../../src/services/agent-service'
import {DEFAULT_PORT} from '../../src/services/agent-service-constants'
import {captureStdOut} from '../helpers/stdout'
const expect = chai.expect
describe('Exec', () => {
xdescribe('#run', () => {
const sandbox = sinon.createSandbox()
function AgentServiceStub(): AgentService {
const agentService = AgentService.prototype as AgentService
sandbox.stub(agentService, 'start')
const start = new Exec([], '') as Exec
sandbox.stub(start, 'agentService').returns(agentService)
return agentService
}
beforeEach(() => {
nock(/localhost/).get('/percy/healthcheck').reply(200)
})
afterEach(() => {
nock.cleanAll()
sandbox.restore()
})
it('starts and stops percy', async () => {
const agentServiceStub = AgentServiceStub()
const stdout = await captureStdOut(async () => {
await Exec.run(['--', 'echo', 'hello'])
})
expect(agentServiceStub.start).to.calledWithMatch(DEFAULT_PORT)
expect(stdout).to.match(/\[percy\] percy has started on port \d+./)
})
xit('starts percy on a specific port')
xit('warns when percy is already running')
})
})
| 41c94a6fd8a1dfa8b3c28a11ede1d84d452da9b2 | [
"TypeScript"
] | 4 | TypeScript | staffe/percy-agent | 79c38f0a5bac42074cf9a99b9a9eda6055e547e1 | ba1eaa76812d3119105f7e67a36988bf43808923 | |
refs/heads/master | <repo_name>ya5e2n/PythonListOverlap<file_sep>/ListOverlap.py
import random
a = []
for x in range(1, random.randint(1, 100)):
a.append(random.randint(1, 100))
b = []
for x in range(1, random.randint(1, 100)):
b.append(random.randint(1, 100))
overlap = []
for x in a:
if x in b:
if x in overlap:
continue
else:
overlap.append(x)
print("Random list 1: \n", a, "\n")
print("Random list 2: \n", b)
print("\nThe elements in common are:\n", overlap)
| f146d9f9032b8ddfcb5677b9083dd504d7868ff9 | [
"Python"
] | 1 | Python | ya5e2n/PythonListOverlap | cfbbb131a0a0bad8f9f2b27275a2a3c13bdb19ff | dd876c9fcda255b87d3a856d61f56668347e9320 | |
refs/heads/master | <repo_name>nathanmousa/ruby-enumerables-introduction-to-map-and-reduce-lab-uci-online-web9-pt-093019<file_sep>/lib/my_code.rb
def map_to_negativize(source_array)
source_array.map { |x| x * -1 }
end
def map_to_no_change(source_array)
source_array.map { |x| x * 1 }
end
def map_to_double(source_array)
source_array.map { |x| x * 2 }
end
def map_to_square(source_array)
source_array.map { |x| x ** 2 }
end
def reduce_to_total(source_array, starting_point=0)
total = starting_point
counter = 0
while counter < source_array.size do
total += source_array[counter]
counter += 1
end
total
end
def reduce_to_all_true(source_array)
i = 0
while i < source_array.length do
return false if !source_array[i]
i += 1
end
return true
end
def reduce_to_any_true(source_array)
i = 0
while i < source_array.length do
return true if source_array[i]
i += 1
end
return false
end | 08d9ad25eab6a728eef4a8399d2977aa13f74a85 | [
"Ruby"
] | 1 | Ruby | nathanmousa/ruby-enumerables-introduction-to-map-and-reduce-lab-uci-online-web9-pt-093019 | f8d93e58dc86eaf42847c9942cec403a9a25698a | 80ee1ac063ab4d5f62c1c1b61723b74f02d1fb54 | |
refs/heads/master | <file_sep># For loops
# z = range(1, 5)
# for x in z:
# print(x)
# While Loop
# x = 0
# while x < 5:
# print(x)
# x += 1
x = 0
while True: # Infinite Loop
if x > 4:
break
print(x)
x += 1
<file_sep>name = 'Abid'
age = 25
salary = 15.5 # Earning in Ks
print(name, age, salary)
print(' My name is ' + name + ' and my age is ' + str(age) + ' and I earn ' + str(salary) + 'K per month')
<file_sep>"""
List, Tuples, Dicts
"""
# List
# l = [1, 5, 2, 19, 5, 25]
# ls = ['A', 'Ad', 'AD']
# l.append(51)
# l.append(11)
# print(l)
# l.sort()
# f = l.pop()
# print(f)
# print(l)
# # Tuples
# t = (1, 4, 5)
# t[1] = 99
# print(t)
#
# import time
# print(time.localtime())
# Dict
d = {}
d = {'name': 'Adnan', 'Education': 'BS', 'Height': 5.8}
person = []
person.append({'name': 'Adnan', 'Education': 'BS', 'Height': 5.8})
person.append({'name': 'Ali', 'Education': 'MA', 'Height': 4.8})
for p in person:
print(p['name'], p['Education'])
#
#
# t = (1, 2)
# l = [1, 3, 4, 5, 1, 25, 13]
# print(time.localtime())
# l.reverse()
# print(l)
<file_sep>def f1():
print("Hi")
def f2(name):
print('Hi ' + name)
def f3(name):
return name
def f4():
return 1, 2
x, x1 = f4()
print(x)
print(x1)
<file_sep>salary = 20.5
age = 30
name = 'Abid'
if salary > 15.5 and age > 20 and name == 'Abid':
print('Wow you earn a lot')
else:
print('Umm Sorry. Work Hard!')
<file_sep>try:
a = 4 / 0
except Exception as ex:
print(str(ex))
finally:
print('Thats all Folks')
<file_sep># file_read = open('LICENSE')
# #print(file_read.read())
# print(len(file_read.readlines()))
write = open('myfile.txt', 'a')
write.write('Hi there\n')
write.close()
<file_sep>a = '<NAME>'
# print(len(a))
# print(a[0:1])
# print(a[2:5])
# b = 'Hi '
# print(b * 3)
multi = """
Hello
Hi
How are you doing today
"""
salary = 300
a = 'Hi I am Jon and I earn ' + str(salary) + ' per month'
a = 'Hi I am {0} and I earn {1} per month'.format('Jon', salary)
print(a)
<file_sep>import sys
args = sys.argv
if len(args) != 2:
print('This is not the correct format')
else:
number = int(args[1])
if number < 10:
print(' It is less than 10')
else:
print('Something else')
<file_sep># Printing Name
a = 'Adnan'
print(a)
| 60427fce08a3ec2f93739a3c805b2ee16a0c8695 | [
"Python"
] | 10 | Python | kadnan/python-fundamentals | 154dad34328b6e9333096159c4041eabf454ca11 | a70041e31c690e96a613c688be709a0c384783da | |
refs/heads/main | <repo_name>tato-gh/phoenix-blue-green<file_sep>/deploy.sh
#!/bin/bash
# e.g.
# deploy.sh service/service-0.1.0+d853862.tar.gz alpha
# - 引数1のファイル(tarボール)をalpha/betaのいずれかにデプロイする
RELEASEFILE=$1
RELEASETARGET=$2
rm -rf ${RELEASETARGET}/*
tar zxf ${RELEASEFILE} -C ${RELEASETARGET}/
docker-compose restart $RELEASETARGET
<file_sep>/README.md
Phoenixアプリケーションを対象として想定した、
DockerコンテナでのBlue-Green Deploymentの習作です。
deploy.sh: alpha/betaフォルダへのtarボール展開
primary.sh: alpha/betaのいずれかをprimaryに設定 (指定しなかった方がtrial)
**NOTE**
- trialのほうはBasic認証がかかるように(とりあえず)している
- 実際の運用時はHTTPSだろうのでその点でのnginxの追加設定は必要
<file_sep>/primary.sh
#!/bin/bash
# e.g.
# primary.sh beta
# - 指定した alpha/beta のいずれかをprimaryに変更する
# - 雑だけれど用意済みの設定ファイルで上書きする
cp nginx/$1.conf nginx/default.conf
docker-compose exec web nginx -s reload
| e78e8a7bdc0cdbe511c5ade5c2030f3fc15046bd | [
"Markdown",
"Shell"
] | 3 | Shell | tato-gh/phoenix-blue-green | 8f44f43050895c6749b9b252f1009646e74692d6 | cc0e06fa84bed905fbf42438caebf0c94228b63e | |
refs/heads/master | <repo_name>gurka/TibiaTools<file_sep>/tibiatools/dat.py
"""Functions to read and parse the Tibia data (Tibia.dat) file.
At the moment only the Tibia 7.1 data file is supported.
"""
from ._utils import readU8, readU16, readU32
def parseDatFile(filename):
"""Opens and parses the given Tibia data file.
Currently only reads items.
Returns a dict with all items, where the key is the item's id
and the value is a dict with the following key-value pairs:
'id': The item's id.
'flags': A dict where the key is the flag and the value is a
list of additional values for the flag.
'width': The item's width (in number of sprites).
'height': The item's height (in number of sprites).
'exactSize': If width or height is greater than 1 this value
will be the item's exact size (in number of pixels),
otherwise it will be None.
'TODO': If this value is set, is the item drawn with
exactSize * exactSize pixels (i.e. a square), or is
this value the larger or smaller side of the item?
'noLayers': The item's number of layers.
'patternWidth': The item's number of patterns (x-axis).
'patternHeight': The item's number of patterns (y-axis).
'noPhases': The item's number of phases (animations).
'sprites': A list of all sprite ids for the item. The length of
this list will be width * height * noLayers *
patternWidth * patternHeight * noPhases.
"""
items = dict()
with open(filename, "rb") as f:
checksum = readU32(f)
print(checksum)
noItems = readU16(f)
readU16(f) # Number of outfits
readU16(f) # Number of effects
readU16(f) # Number of distances (TODO: better name)
# First item has id 100, so iterate from 100 to 100 + noItems
for itemId in range(100, 100 + noItems):
flags = dict()
flag = readU8(f)
while flag != 0xFF:
if flag == 0x00:
speed = readU16(f)
flags[flag] = [speed]
elif flag == 0x10:
level = readU16(f)
color = readU16(f)
flags[flag] = [level, color]
elif flag in [0x07, 0x08]:
maxLength = readU16(f)
flags[flag] = [maxLength]
elif flag in [0x13, 0x16]:
offset = readU16(f)
flags[flag] = [offset]
elif flag == 0x1A:
value = readU16(f)
flags[flag] = [value]
else:
flags[flag] = []
flag = readU8(f) # Next flag
# Width and height is the number of sprites (x and y axis) this item is drawn with
# width=1, height=1 is drawn with 1 sprite, which means 32x32 pixels
# width=2, height=2 is drawn with 4 sprites, which means 64x64 pixels
width = readU8(f)
height = readU8(f)
# Exact size is used when an item with width > 1 or height > 1 is smaller than
# the actual sprites size
# I.e. an item with width=2, height=2 can have exactSize=40
# It is drawn using 4 sprites but only 40x40 pixels needs to be drawn
if width > 1 or height > 1:
exactSize = readU8(f)
else:
exactSize = None
# Multiple layers are used when an item is drawn using multiple sprites
# at the same position
noLayers = readU8(f)
# Pattern width and height are used when multiple items with the same id are
# drawn besides eachother, to make them blend together better
# If grass (let's say itemId = 1) is drawn in a 4x4 pattern, the map might have:
# 1111
# 1111
# But grass has patternWidth=4, patternHeight=4,
# so the sprites used to draw the grass are:
# 1234
# 5678
# If the item is stackable and has more than 8 sprites,
# then patternWidth=4 and patternHeight=2 and "count" is used to
# choose which sprite to draw
patternWidth = readU8(f)
patternHeight = readU8(f)
# Phases are used to animate items, the phase to choose is based on
# current time / game step:
# currentPhase = gamestep % noPhases
noPhases = readU8(f)
# TODO: The below is probably not 100% correct
# Draw order and read order is probably the same...
# If the item has width=2 and height=2 there will be 4 sprites to draw:
# AB
# CD
# They needs to be drawn in order D, C, B, A, and is probably read in
# this order as well.
# The sprites are read in a specific order. If we have an item with:
# width=2, height=2, noLayers=2, patternWidth=2, patternHeight=2, noPhases=2
# Then the order is:
# phase 0 - patternHeight 0 - patternWidth 0 - layer 0 - height 0 - width 0
# phase 0 - patternHeight 0 - patternWidth 0 - layer 0 - height 0 - width 1
# phase 0 - patternHeight 0 - patternWidth 0 - layer 0 - height 1 - width 0
# phase 0 - patternHeight 0 - patternWidth 0 - layer 0 - height 1 - width 1
# phase 0 - patternHeight 0 - patternWidth 0 - layer 1 - height 0 - width 0
# phase 0 - patternHeight 0 - patternWidth 0 - layer 1 - height 0 - width 1
# phase 0 - patternHeight 0 - patternWidth 0 - layer 1 - height 1 - width 0
# phase 0 - patternHeight 0 - patternWidth 0 - layer 1 - height 1 - width 1
# and so on...
# So the get the spriteId for
# phase=1, pattern width=1, pattern height=1, layer=0, height=1, width=0:
# index = 1 * patternHeight * patternWidth * layer * height * width
# index += 1 * patternWidth * layer * height * width
# index += 0 * layer * height * width
# index += 0 * height * width
# index += 1 * width
# index += 0
# spriteId = sprites[index]
# The order to draw part of an item (each sprite) is important
# If the item has width = 2 and height = 2 the item is drawn using 4 sprites:
# 12
# 34
# And we need to draw them in the order 4, 3, 2, 1
sprites = []
for sprite in range(noPhases * patternHeight * patternWidth *
noLayers * height * width):
sprites.append(readU16(f))
items[itemId] = {
'id': itemId,
'flags': flags,
'width': width,
'height': height,
'exactSize': exactSize,
'noLayers': noLayers,
'patternWidth': patternWidth,
'patternHeight': patternHeight,
'noPhases': noPhases,
'sprites': sprites,
}
return items
def flagToString(flag, extras=[]):
"""Converts the given flag to a string that describes it.
The optional argument extra should be a list of any additional
values for this flag.
"""
if flag == 0x00:
return "isGround - Speed: {}".format(extras[0])
elif flag == 0x01:
return "isOnTop"
elif flag == 0x02:
return "walkThrough"
elif flag == 0x03:
return "isContainer"
elif flag == 0x04:
# If sprites == 8 (patternWidth: 4, patternHeight: 2)
# Then sprites => count
return "isStackable"
elif flag == 0x05:
# Right-click -> use with crosshair
return "isUsable"
elif flag == 0x06:
# Only one item... (1124)
return "ladderUp"
elif flag == 0x07:
return "isWriteable - Max length: {}".format(extras[0])
elif flag == 0x08:
return "isReadable - Max length: {}".format(extras[0])
elif flag == 0x09:
return "isFluidContainer"
elif flag == 0x0A:
return "isSplash"
elif flag == 0x0B:
return "isBlocking"
elif flag == 0x0C:
return "isImmovable"
elif flag == 0x0D:
return "blocksMissile"
elif flag == 0x0E:
return "blocksCreature"
elif flag == 0x0F:
return "isEquipable"
elif flag == 0x10:
return "hasLight - Light level: {} Light color: {}".format(extras[0], extras[1])
elif flag == 0x11:
return "canSeeUnder"
elif flag == 0x12:
return "noLevelChange"
elif flag == 0x13:
return "drawOffset - Offset: {}".format(extras[0])
elif flag == 0x14:
return "playerColorTemplates"
elif flag == 0x15:
# No item with this flag
return "unknown (0x15)"
elif flag == 0x16:
return "offsetLifebar - Offset: {}".format(extras[0])
elif flag == 0x17:
# No item with this flag
return "unknown (0x17)"
elif flag == 0x18:
return "noDecay"
elif flag == 0x19:
return "animationWhenIdle"
elif flag == 0x1A:
# Right-click -> Use
return "canDoAction - Value: {}".format(extras[0])
else:
return "unknown (0x{:02X})".format(flag)
<file_sep>/dat_test.py
#!/usr/bin/env python3
from tibiatools import dat
if __name__ == '__main__':
items = dat.parseDatFile("Tibia.dat")
for itemId in items:
print(itemId)
<file_sep>/tibiatools/_utils.py
"""Internal functions used by tibiatools"""
def readU8(f):
"""Reads an unsigned 8 bit integer from file object `f`."""
return int.from_bytes(f.read(1), byteorder='little', signed=False)
def readU16(f):
"""Reads an unsigned 16 bit integer from file object `f`."""
return int.from_bytes(f.read(2), byteorder='little', signed=False)
def readU32(f):
"""Reads an unsigned 32 bit integer from file object `f`."""
return int.from_bytes(f.read(4), byteorder='little', signed=False)
| 8daa967508d69c635c42d72d640b0f10d644b929 | [
"Python"
] | 3 | Python | gurka/TibiaTools | 16677c962a4199ebf8e695214d7a6fea56c91ceb | c894ea25c36beb68c834efdcb55f153f0ce616a6 | |
refs/heads/master | <file_sep>from flask import Flask
import subprocess
from flask import jsonify
#from squid_py.ddo.metadata import Metadata
#from squid_py.ocean import Ocean
#from squid_py.config import Config
from smbus import SMBus
# Import basic packages
import io
import logging
import os
import glob
import warnings
warnings.filterwarnings('ignore')
from time import gmtime, strftime
# Imports the Google Cloud client library
from google.cloud import vision
from google.cloud.vision import types
# Instantiates a client
client = vision.ImageAnnotatorClient()
# Path storing Pi camera photos
img_path = './images'
# Classification using Google Vision API
def proccess_picture():
all_results = list()
for img in os.listdir(img_path):
if img.endswith('.jpg'):
image = os.path.join(img_path, img)
logging.info(image)
with io.open(image, 'rb') as image_file:
content = image_file.read()
image = types.Image(content=content)
response = client.label_detection(image=image)
labels = response.label_annotations
logging.info(strftime('%Y-%m-%d %H:%M:%S', gmtime()))
logging.info('Labels:')
result = []
for label in labels:
logging.info(label)
result.append(label.description)
all_results.append(result)
return all_results
app = Flask(__name__)
bus = SMBus(1)
#ocean = Ocean(Config(filename='config.ini'))
@app.route("/api/status", methods=['GET'])
def status():
return "status"
@app.route("/api/snap", methods=['GET'])
def snap():
#subprocess.call('flask_app/scripts/take_photos.sh')
#subprocess.call('/home/pi/git/pines-and-electronics/flask_app/scripts/take_photos.sh')
this_cmd = "raspistill -t 10000 -tl 2000 -o /home/pi/git/pines-and-electronics/flask_app/images/image%04d.jpg"
#this_cmd = "raspistill -o cam.jpg"
#this_cmd = "ls"
subprocess.call(this_cmd, shell=True)
print("Ran", this_cmd)
return 'snap!' + this_cmd
@app.route("/api/register", methods=['GET'])
def register():
label_list = proccess_picture()
summary = list()
for i, label in enumerate(label_list):
print(i, label)
summary.append("|".join(label))
#metadata =Metadata.get_example()
#metadata['base']['tags'] = labels
#ddo = ocean.assets.create(metadata, ocean.accounts._accounts[0])
#return ddo.did
return " *** ".join(summary)
@app.route("/api/snapshot", methods=['GET'])
def snapshot():
return "snapshot"
@app.route("/api/linear", methods=['POST'])
def linear():
bus.write_byte(8, ord("S"))
return "linear"
@app.route("/api/start", methods=['GET'])
def start():
bus.write_byte(8, ord("S"))
return "START"
@app.route("/api/stop", methods=['GET'])
def stop():
bus.write_byte(8, ord("s"))
return "STOP"
@app.route("/api/steer", methods=['POST'])
def steer():
bus.write_byte(9, ord('x'))
return "steer"
# def publish_asset():
# return ocean.assets.create(Metadata.get_example())
if __name__ == "__main__":
app.run(port=3333)
<file_sep>#!/usr/bin/env python
# -*- coding: utf-8 -*-
"""The setup script."""
from setuptools import find_packages, setup
with open('README.md') as readme_file:
readme = readme_file.read()
install_requirements = [
'coloredlogs==10.0',
'Flask==1.0.2',
'Flask-Cors==3.0.6',
'flask-swagger==0.2.14',
'flask-swagger-ui==3.20.9',
'gunicorn==19.9.0',
'PyYAML==5.1',
'pytz==2018.5',
'squid-py==0.5.14',
'absl-py == 0.7.1',
'astor == 0.7.1',
'cachetools == 3.1.0',
'chardet == 3.0.4',
'gast == 0.2.2',
'google-api-core == 1.9.0',
'google-api-python-client == 1.7.8',
'google-auth== 1.6.3',
'google-auth-httplib2 == 0.0.3',
'google-cloud-core == 0.29.1',
'google-cloud-storage == 1.14.0',
'google-cloud-vision == 0.36.0',
'google-resumable-media == 0.3.2',
'googleapis-common-protos == 1.5.9',
'grpcio == 1.19.0',
'httplib2 == 0.12.1',
'idna == 2.8',
#'keras-applications == 1.0.7',
#'keras-preprocessing == 1.0.9',
'markdown == 3.1',
'mock == 2.0.0',
'pbr == 5.1.3',
'protobuf == 3.7.1',
'pyasn1 == 0.4.5',
'pyasn1-modules == 0.2.4',
'requests == 2.21.0',
'rsa == 4.0',
#'tensorboard == 1.13.1',
#'tensorflow == 1.13.1',
#'tensorflow-estimator == 1.13.0',
'termcolor == 1.1.0',
'uritemplate == 3.0.0',
'urllib3 == 1.24.1',
'werkzeug == 0.15.2',
'smbus-cffi == 0.5.1'
]
setup_requirements = ['pytest-runner==2.11.1', ]
dev_requirements = [
'bumpversion==0.5.3',
'pkginfo==1.4.2',
'twine==1.11.0',
# not virtualenv: devs should already have it before pip-installing
'watchdog==0.8.3',
]
test_requirements = [
'codacy-coverage==1.3.11',
'coverage==4.5.1',
'mccabe==0.6.1',
'pylint==2.2.2',
'pytest==3.4.2',
'tox==3.2.1',
]
setup(
author="leucothia",
classifiers=[
'Development Status :: 2 - Pre-Alpha',
'Intended Audience :: Developers',
'License :: OSI Approved :: Apache Software License',
'Natural Language :: English',
'Programming Language :: Python :: 3.6',
'Programming Language :: Python :: 3.7',
],
description="🚗 Car backend.",
extras_require={
'test': test_requirements,
'dev': dev_requirements + test_requirements,
},
include_package_data=True,
install_requires=install_requirements,
keywords='pines backend',
license="Apache Software License 2.0",
long_description=readme,
long_description_content_type="text/markdown",
name='car-backend',
packages=find_packages(include=['flask_app', 'flask_app.myapp', 'camera']),
setup_requires=setup_requirements,
test_suite='tests',
tests_require=test_requirements,
url='https://github.com/odysseyhack/pines-and-electronics',
version='0.1.0',
zip_safe=False,
)
<file_sep># Take photos using RPI camera
#raspistill -t 10000 -tl 2000 -o ./images/
raspistill -t 10000 -tl 2000 -o /home/pi/git/pines-and-electronics/flask_app/images/image%04d.jpg
<file_sep>import pytest
from flask_app.flask_app.myapp import app
app = app
@pytest.fixture
def client():
client = app.test_client()
yield client<file_sep>from flask_app.camera.gcp_vision_multi_img import proccess_picture
# def test_status(client):
# assert client.get('/api/status').data == b"status"
# # client.get('/api/snap')
#
def test_register_in_ocean(client):
client.get('/api/register')
# def test_process_pictures():
# result = proccess_picture()<file_sep># Import basic packages
import io
import logging
import os
import glob
import warnings
warnings.filterwarnings('ignore')
from time import gmtime, strftime
# Imports the Google Cloud client library
from google.cloud import vision
from google.cloud.vision import types
# Instantiates a client
client = vision.ImageAnnotatorClient()
# Path storing Pi camera photos
img_path = './images'
# Classification using Google Vision API
def proccess_picture():
for img in os.listdir(img_path):
if img.endswith('.jpg'):
image = os.path.join(img_path, img)
logging.info(image)
with io.open(image, 'rb') as image_file:
content = image_file.read()
image = types.Image(content=content)
response = client.label_detection(image=image)
labels = response.label_annotations
logging.info(strftime('%Y-%m-%d %H:%M:%S', gmtime()))
logging.info('Labels:')
result = []
for label in labels:
logging.info(label)
result.append(label.description)
return result
<file_sep>from backend.car.car_controller import CarController
def test_car_controller():
car = CarController()
send_command = car.send_command('status')
assert send_command
filter = car.contract.events.CarCommandSent.createFilter(fromBlock=0, toBlock='latest')
logs = filter.get_all_entries()
assert len(logs) > 0
event = car.subscribe_to_command_event(
20,
car.log_event(car.COMMAND_SENT_EVENT),
(),
wait=True
)
assert event, 'no event for command_sent_event'
assert car.parse_command(logs[-1]) == "status"
<file_sep>from backend.car.car_controller import CarController
class main():
car = CarController()
last_block_number = 0
while True:
filter = car.contract.events.CarCommandSent.createFilter(fromBlock=0, toBlock='latest')
logs = filter.get_all_entries()
block_number = logs[-1].blockNumber
if block_number != last_block_number:
car.execute_command(car.parse_command(logs[-1]))
last_block_number= block_number
if __name__ == '__main__':
main()<file_sep>import json
import logging
import requests
from car.event_listener import EventListener
from squid_py.config import Config
from squid_py.config_provider import ConfigProvider
from squid_py.keeper.web3_provider import Web3Provider
from web3.contract import ConciseContract
class CarController:
CONTRACT_NAME = 'CarController'
def __init__(self):
self.config = Config('config.ini')
self.COMMAND_SENT_EVENT = 'CarCommandSent'
ConfigProvider.set_config(self.config)
@property
def contract(self):
return Web3Provider.get_web3().eth.contract(
address=self.config.get('resources', 'car.contract.address'),
abi=self.get_abi('artifacts/CarController.json')['abi']
)
@property
def contract_concise(self):
return ConciseContract(Web3Provider.get_web3().eth.contract(
address=self.config.get('resources', 'car.contract.address'),
abi=self.get_abi('artifacts/CarController.json')['abi']
))
@staticmethod
def get_abi(path):
with open(path) as f:
contract_dict = json.loads(f.read())
return contract_dict
def send_command(self, command):
"""
:param command:
:return:
"""
logging.info(f'Sending commnad {command} to the car.')
return self.contract.functions.sendCommand(command).transact()
def subscribe_to_event(self, event_name, timeout, event_filter, callback=False,
timeout_callback=None, args=None, wait=False):
return EventListener(
self.contract,
event_name,
args,
filters=event_filter
).listen_once(
callback,
timeout_callback=timeout_callback,
timeout=timeout,
blocking=wait
)
def subscribe_to_command_event(self, timeout, callback, args, wait=False):
return self.subscribe_to_event(
self.COMMAND_SENT_EVENT,
timeout,
# {'_command': 'Forward'},
None,
callback=callback,
args=args,
wait=wait
)
@staticmethod
def log_event(event_name):
def _process_event(event):
print(f'Received event {event_name}: {event}')
return _process_event
def parse_command(self, event):
if event is None:
logging.error('No event to parse.')
if event.args is None:
logging.info('There are no commnads in this event')
command = event.args._command
return command
def execute_command(self, command):
logging.info(f'Calling command:{command}')
if command.startswith('status'):
requests.get(f'{self.config.get("resources","raspberry.url")}/api/status')
elif command.startswith('snap'):
requests.get(f'{self.config.get("resources", "raspberry.url")}/api/snap')
elif command.startswith('register'):
requests.post(f'{self.config.get("resources", "raspberry.url")}/api/register')
elif command.startswith('snapshot'):
requests.get(f'{self.config.get("resources", "raspberry.url")}/api/snapshot')
elif command.startswith('linear'):
requests.get(f'{self.config.get("resources", "raspberry.url")}/api/linear')
elif command.startswith('steer'):
requests.get(f'{self.config.get("resources", "raspberry.url")}/api/steer')
else:
logging.error(f'Invalid command: {command}')
# def do_request(self):
# try:
# requests.get(f'{self.config.get("resources", "raspberry.url")}/api/snapshot')
# except:
<file_sep>> 
<h1 align="center">Pines & Electronics</h1>
> 🕹🚗 Hacking in the Nature2.0 track.
---
## Table of Contents
- [📄 DAO](#dao)
- [🔙🔚 Backend](#backend)
- [🌿 API](#api)
- [🏛 License](#license)
---
## 📄 DAO
This component has a simple smart contract that emit an event with the command that we want to
send to the robotic car.
First of all we need to compile the contract and generate the abi files:
```bash
npx install
npx truffle compile
```
To deploy the contract we need to run the following commands:
```bash
npx truffle deploy
```
Once this happen we can get the abi files from the build folder to interact with the contracts
and the address of the contract.
## 🔙🔚 Backend
## 🌿 API
## 🏛 License
```text
Copyright 2018
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
```<file_sep>
# Activate conda environment
source activate pines_conda
# Connect to google API and predict
python ./image_demo/gcp_vision_multi_img.py
| 2aeb6687a38735e8bce24767e47eebf30908261c | [
"Markdown",
"Python",
"Shell"
] | 11 | Python | odysseyhack/pines-and-electronics | 82465582fd24bbb249215bdea23ff853fab0b1b4 | bd545bb025b3033523b39c616a6d74b8c96bb3c0 | |
refs/heads/master | <repo_name>Qix-/starfuse<file_sep>/starfuse/pak/sbbf03.py
"""Implementation of the StarBound block file v2/3 storage"""
import logging
import struct
from starfuse.fs.mapped_file import MappedFile
log = logging.getLogger(__name__)
class InvalidMagic(Exception):
"""A block file has an invalid magic string"""
def __init__(self, path):
super(InvalidMagic, self).__init__('a block file has an invalid magic string: %s' % path)
class SBBF03(MappedFile):
"""Implements a StarBound block file v3 store that is backed by a file
Can also be used to read in v2.
It's worth noting that the memory regions in this class are mapped and not
read-in."""
def __init__(self, path, page_count, read_only=False):
super(SBBF03, self).__init__(path, page_count, read_only=read_only)
self._header_size = 0
self._block_size = 0
self.header = None
self.user_header = None
self.blocks = dict()
self.__load(path)
def __del__(self):
self.close()
def block_region(self, bid):
"""Gets a block region given the block ID"""
base_offset = self._header_size + (self._block_size * bid)
return self.region(offset=base_offset, size=self._block_size)
@property
def block_count(self):
block_region_size = len(self) - self._header_size
return block_region_size // self._block_size
def __load(self, path):
log.debug('loading SBBF03 block file: %s', path)
region = self.region(0, 32)
# magic constant
magic = region.read(6)
if magic not in [b'SBBF03', b'SBBF02']:
raise InvalidMagic(path)
log.debug('block file has valid magic constant: %s', magic)
# get the header and block size
# this is all we need to actually read from the file before we start mmap-ing.
# this is because we want to be able to mmap the header as well, and all we need to know
# are the header sizes and block sizes.
(self._header_size, self._block_size) = struct.unpack('>ii', region.read(8))
log.debug('header_size=%d, block_size=%d', self._header_size, self._block_size)
# calculate number of blocks
log.debug('block count: %d', self.block_count)
# map header
self.header = self.region(offset=0, size=self._header_size)
self.user_header = self.header.region(0x20)
# map user header
self.user_header = self.header.region(offset=0x20)
log.debug('mapped headers successfully')
<file_sep>/starfuse/main.py
"""StarFuse, the StarBound packfile FUSE filesystem
This program allows you to mount StarBound PAK files as a directory,
granting you the ability to work directly within the PAK file instead
of having to unpack/re-pack it over and over again.
"""
import logging
import starfuse.config as config
from fuse import FUSE
from starfuse.fusepak import FusePAK
log = logging.getLogger(__name__)
def main():
"""StarFuse entry point"""
log.info('starting StarFuse')
log.info('mounting pakfile %s as %s', config.pak_file, ('read-only' if config.read_only else 'read/write'))
pak = FusePAK(config.pak_file, page_count=config.page_count, read_only=config.read_only)
log.info('mounting on %s', config.mount_dir)
FUSE(pak, config.mount_dir, foreground=True)
<file_sep>/starfuse/pak/btreedb4.py
"""
BTreeDB4 implementation
Heavily influenced by Blixt's starfuse-py utilities,
this implementation's sine qua non.
"""
import binascii
import bisect
import io
import struct
import logging
import starfuse.pak.sbon as sbon
from starfuse.pak.sbbf03 import SBBF03
# Override range with xrange when running Python 2.x.
try:
range = xrange
except:
pass
log = logging.getLogger(__name__)
class BTreeKeyError(Exception):
def __init__(self, key):
super(BTreeKeyError, self).__init__(key)
def keyed(fn):
def wrapper(*args, **kwargs):
raw_key = False
key = None
try:
if 'raw_key' in kwargs:
raw_key = kwargs['raw_key']
del kwargs['raw_key']
self = args[0]
key = args[1]
if not raw_key:
key = self.encode_key(key)
assert len(key) == self.key_size, 'Invalid key length'
nargs = (self, key) + args[2:]
return fn(*nargs, **kwargs)
except (BTreeKeyError, KeyError):
hex_key = binascii.hexlify(key)
if raw_key:
raise BTreeKeyError(hex_key)
else:
raise BTreeKeyError('%s (%s)' % (hex_key, args[1]))
return wrapper
class BTreeDB4(SBBF03):
"""A B-tree database format on top of the SBBF03 block format.
Note: The developers of this format probably intended for the underlying
file format to be arbitrary, but this database has pretty strong
connections to SBBF02 right now so it's been implemented as inheriting from
that file format. In the future we may want to split away from the
inheritance chain and instead use the SBBF02 file as an API.
"""
def __init__(self, path, page_count, read_only=False):
super(BTreeDB4, self).__init__(path, page_count, read_only=False)
self.key_size = None
# Set this attribute to True to make reading more forgiving.
self.repair = False
self.alternate_root_node = None
self.root_node = None
self.root_node_is_leaf = None
self.__load()
def encode_key(self, key):
"""Can be overridden to encode a key before looking for it in the
database (for example if the key needs to be hashed)."""
return key
def block(self, index):
"""Gets a block object given the specified index"""
region = self.block_region(index)
signature = bytes(region.read(2))
if signature in _block_types:
return _block_types[signature](self, index, region)
if signature is not b'\0\0':
raise Exception('Invalid signature detected: %s', signature)
return None
@keyed
def _leaf_for_key(self, key):
"""Returns the binary data for the provided key."""
block = self.block(self.root_node)
assert block is not None, 'Root block is None'
# Scan down the B-tree until we reach a leaf.
while isinstance(block, BTreeIndex):
block_number = block.block_for_key(key)
block = self.block(block_number)
assert isinstance(block, BTreeLeaf), 'Did not reach a leaf'
return block
@keyed
def file_contents(self, key):
leaf = self._leaf_for_key(key, raw_key=True)
stream = LeafReader(self, leaf)
# The number of keys is read on-demand because only leaves pointed to
# by an index contain this number (others just contain arbitrary data).
num_keys, = struct.unpack('>i', stream.read(4))
assert num_keys < 1000, 'Leaf had unexpectedly high number of keys'
for i in range(num_keys):
cur_key = stream.read(self.key_size)
# TODO do some smarter parsing here (read_bytes is easy to recreate
# using regions)
value = sbon.read_bytes(stream)
if cur_key == key:
return value
raise BTreeKeyError(key)
@keyed
def file_size(self, key):
leaf = self._leaf_for_key(key, raw_key=True)
stream = LeafReader(self, leaf)
# The number of keys is read on-demand because only leaves pointed to
# by an index contain this number (others just contain arbitrary data).
num_keys, = struct.unpack('>i', stream.read(4))
assert num_keys < 1000, 'Leaf had unexpectedly high number of keys'
for i in range(num_keys):
cur_key = stream.read(self.key_size)
# TODO do much better streaming here when LeafReader is refactored
size = sbon.read_varlen_number(stream)
stream.read(size)
if cur_key == key:
return size
raise BTreeKeyError(key)
def __load(self):
stream = self.user_header
# Require that the format of the content is BTreeDB4.
db_format = sbon.read_fixlen_string(stream, 12)
assert db_format == 'BTreeDB4', 'Expected binary tree database'
log.debug('found BTreeDB4 header successfully')
# Name of the database.
self.identifier = sbon.read_fixlen_string(stream, 12)
log.info('database name: %s', self.identifier)
fields = struct.unpack('>i?xi?xxxi?', stream.read(19))
self.key_size = fields[0]
log.debug('key size=%d', self.key_size)
# Whether to use the alternate root node index.
self.alternate_root_node = fields[1]
if self.alternate_root_node:
self.root_node, self.root_node_is_leaf = fields[4:6]
self.other_root_node, self.other_root_node_is_leaf = fields[2:4]
else:
self.root_node, self.root_node_is_leaf = fields[2:4]
self.other_root_node, self.other_root_node_is_leaf = fields[4:6]
log.debug('loaded root nodes: root=%d isleaf=%r', self.root_node, self.root_node_is_leaf)
class BTreeBlock(object):
def __init__(self, btree, index, region):
self._btree = btree
self.index = index
self.block_region = region
class BTreeIndex(BTreeBlock):
SIGNATURE = b'II'
__slots__ = ['keys', 'level', 'num_keys', 'values']
def __init__(self, btree, index, region):
super(BTreeIndex, self).__init__(btree, index, region)
self.level, self.num_keys, left_block = struct.unpack('>Bii', self.block_region.read(9))
self.keys = []
self.values = [left_block]
for i in range(self.num_keys):
key = self.block_region.read(btree.key_size)
block, = struct.unpack('>i', self.block_region.read(4))
self.keys.append(key)
self.values.append(block)
def __str__(self):
return 'Index(level={}, num_keys={})'.format(self.level, self.num_keys)
def block_for_key(self, key):
i = bisect.bisect_right(self.keys, key)
return self.values[i]
class BTreeLeaf(BTreeBlock):
SIGNATURE = b'LL'
__slots__ = ['data', 'next_block']
def __init__(self, btree, index, region):
super(BTreeLeaf, self).__init__(btree, index, region)
# Substract 6 for signature and next_block.
self.data = self.block_region.read(btree._block_size - 6)
value, = struct.unpack('>i', self.block_region.read(4))
self.next_block = value if value != -1 else None
def __str__(self):
return 'Leaf(next_block={})'.format(self.next_block)
class BTreeFree(BTreeBlock):
SIGNATURE = b'FF'
__slots__ = ['next_free_block']
def __init__(self, btree, index, region):
super(BTreeFree, self).__init__(btree, index, region)
self.raw_data = self.block_region.region()
value, = struct.unpack('>i', self.raw_data[:4])
self.next_free_block = value if value != -1 else None
def __str__(self):
return 'Free(next_free_block={})'.format(self.next_free_block)
class BTreeRestoredLeaf(BTreeLeaf):
def __init__(self, free_block):
assert isinstance(free_block, BTreeFree), 'Expected free block'
self.data = free_block.raw_data[:-4]
value, = struct.unpack('>i', free_block.raw_data[-4:])
self.next_block = value if value != -1 else None
def __str__(self):
return 'RestoredLeaf(next_block={})'.format(self.next_block)
class LeafReader(object):
"""A pseudo-reader that will cross over block boundaries if necessary."""
__slots__ = ['_file', '_leaf', '_offset', '_visited']
def __init__(self, file, leaf):
assert isinstance(file, BTreeDB4), 'File is not a BTreeDB4 instance'
assert isinstance(leaf, BTreeLeaf), 'Leaf is not a BTreeLeaf instance'
self._file = file
self._leaf = leaf
self._offset = 0
self._visited = [leaf.index]
def read(self, length):
offset = self._offset
if offset + length <= len(self._leaf.data):
self._offset += length
return self._leaf.data[offset:offset + length]
buffer = io.BytesIO()
# If the file is in repair mode, make the buffer available globally.
if self._file.repair:
LeafReader.last_buffer = buffer
# Exhaust current leaf.
num_read = buffer.write(self._leaf.data[offset:])
length -= num_read
# Keep moving onto the next leaf until we have read the desired amount.
while length > 0:
next_block = self._leaf.next_block
assert next_block is not None, 'Tried to read too far'
assert next_block not in self._visited, 'Tried to read visited block'
self._visited.append(next_block)
self._leaf = self._file.block(next_block)
if self._file.repair and isinstance(self._leaf, BTreeFree):
self._leaf = BTreeRestoredLeaf(self._leaf)
assert isinstance(self._leaf, BTreeLeaf), \
'Leaf pointed to non-leaf %s after reading %d byte(s)' % (next_block, buffer.tell())
num_read = buffer.write(self._leaf.data[:length])
length -= num_read
# The new offset will be how much was read from the current leaf.
self._offset = num_read
data = buffer.getvalue()
buffer.close()
return data
_block_types = {
BTreeIndex.SIGNATURE: BTreeIndex,
BTreeLeaf.SIGNATURE: BTreeLeaf,
BTreeFree.SIGNATURE: BTreeFree
}
<file_sep>/starfuse/pak/pakfile.py
"""VFS tracked, BTree package backed Pakfile
Note that this implementation supports asset pakfiles (including modpak files)
that are keyed with SHA256 digests for StarBound. It will not work with anything else.
"""
import hashlib
import io
import logging
import starfuse.pak.sbon as sbon
from starfuse.pak.btreedb4 import BTreeDB4
from starfuse.fs.vfs import VFS, FileNotFoundError, IsADirError, NotADirError
log = logging.getLogger(__name__)
class KeyStore(BTreeDB4):
"""A B-tree database that uses SHA-256 hashes for key lookup."""
def encode_key(self, key):
return hashlib.sha256(key.encode('utf-8')).digest()
class Package(KeyStore):
"""A B-tree database representing a package of files."""
DIGEST_KEY = '_digest'
INDEX_KEY = '_index'
def __init__(self, path, page_count, read_only=False):
super(Package, self).__init__(path, page_count, read_only=read_only)
self._index = None
def encode_key(self, key):
return super(Package, self).encode_key(key.lower())
def get_digest(self):
return self.get(Package.DIGEST_KEY)
def get_index(self):
if self._index:
return self._index
# TODO optimize this to use new regions system after refactoring BTreeDB
stream = io.BytesIO(self.file_contents(Package.INDEX_KEY))
if self.identifier == 'Assets1':
self._index = sbon.read_string_list(stream)
elif self.identifier == 'Assets2':
self._index = sbon.read_string_digest_map(stream)
return self._index
class Pakfile(object):
def __init__(self, path, page_count, read_only=False):
self.vfs = VFS()
self.pkg = Package(path, page_count, read_only=False)
log.debug('obtaining file list')
file_index = self.pkg.get_index()
log.debug('registering files with virtual filesystem')
for filepath, lookup in file_index.iteritems():
self.vfs.add_file(filepath, lookup, mkdirs=True)
log.info('registered %d files with virtual filesystem', len(file_index))
@property
def read_only(self):
return self.pkg.read_only
@read_only.setter
def read_only(self, val):
self.pkg.read_only = val
def entry(self, abspath):
if abspath is None or abspath == '/':
return (self.vfs.root, None, self.vfs.root, False)
names = self.vfs._split_path(abspath)
direc = self.vfs._mkdirp(names[:-1], srcpath=abspath, mkdirs=False)
fname = names[-1]
if fname not in direc:
raise FileNotFoundError(abspath)
entry = direc[fname]
# (directory dict, filename, lookup entry, True if file, False if directory)
return (direc, fname, entry, not isinstance(entry, dict))
def directory_listing(self, abspath):
(_, _, lookup, isfile) = self.entry(abspath)
if isfile:
raise NotADirError(abspath)
return lookup.keys()
def file_size(self, abspath):
(_, _, _, isfile) = self.entry(abspath)
if not isfile:
raise IsADirError(abspath)
return self.pkg.file_size(abspath)
def file_contents(self, abspath, offset=0, size=-1):
(_, _, _, isfile) = self.entry(abspath)
if not isfile:
raise IsADirError(abspath)
return self.pkg.file_contents(abspath)[offset:offset + size]
def readdir(self, abspath):
(_, _, lookup, isfile) = self.entry(abspath)
if isfile:
raise NotADirError(abspath)
results = []
for name, lobj in lookup.iteritems():
results.append((name, not isinstance(lobj, dict)))
return results
<file_sep>/README.md
# StarFuse
Mount StarBound .pak files as FUSE filesystems.
This codebase aims to serve as a reference implementation for both reading and writing modern Starbound files.
## Installation
Just want to use StarFuse?
```console
$ pip install starfuse
```
Want to develop StarFuse?
```console
$ git clone https://github.com/qix-/starfuse
$ cd starfuse
$ virtualenv env
$ . env/bin/activate
$ pip install -r requirements.txt
```
# License
Copyright © 2016 by <NAME>. Licensed under the [MIT License](LICENSE.txt) for the StarBound community.
Developers of StarBound and ChuckleFish as a whole may use this software freely both commercially and otherwise (whilst adhering to the MIT License, which doesn't ask for much). I encourage corrections from the team if they so choose.
<file_sep>/setup.py
from distutils.core import setup
setup(
name='starfuse',
packages=['starfuse', 'starfuse.pak', 'starfuse.pak', 'starfuse.fs'],
version='0.3.0',
description='Mount StarBound .pak files as FUSE filesystems',
author='<NAME>',
author_email='<EMAIL>',
url='https://github.com/qix-/starfuse',
download_url='https://github.com/qix-/starfuse/tarball/0.1.0',
keywords=['starfuse', 'starbound', 'fuse', 'pak', 'development', 'mod', 'extension'],
classifiers=[],
install_requires=['fusepy'],
entry_points={
'console_scripts': [
'starfuse = starfuse.main:main'
],
},
)
<file_sep>/starfuse/config.py
"""StarFuse configuration/opt-parsing"""
import sys
import argparse
import logging
LOGGERFMT = '%(asctime)s [%(levelname)s] %(name)s <%(funcName)s>: %(message)s'
class Config(object):
"""StarFuse configuration class and option parser"""
def __init__(self):
parser = argparse.ArgumentParser(
prog='starfuse',
description='Mounts StarBound .pak files as FUSE filesystems')
parser.add_argument('pakfile', type=str, help='the .pak file on which to operate')
parser.add_argument('mount_dir', type=str, help='the directory on which to mount the PAK file')
parser.add_argument('-v', '--verbose', help='be noisy', action='store_true')
parser.add_argument('-w', '--write', help='allow modifications to the .pak file', action='store_true')
parser.add_argument('--pages', type=int, help='map this number of pages at a time (default: 256)', default=256)
self._args = parser.parse_args()
if self._args.verbose:
logging.basicConfig(level=logging.DEBUG, format=LOGGERFMT)
else:
logging.basicConfig(level=logging.INFO, format=LOGGERFMT)
@property
def pak_file(self):
"""Gets the .pak file path to use"""
return self._args.pakfile
@property
def mount_dir(self):
"""Gets the target mount directory"""
return self._args.mount_dir
@property
def page_count(self):
"""Gets the number of pages to map at once"""
return self._args.pages
@property
def read_only(self):
"""Whether or not the file is read-only protected"""
return not self._args.write
sys.modules[__name__] = Config()
<file_sep>/starfuse/fs/vfs.py
"""Virtual File System, which manages paths and their lookups"""
import os
import os.path
import logging
assert os.sep == '/', 'Starfuse cannot be used on non-unix systems'
log = logging.getLogger(__name__)
class NotADirError(Exception):
pass
class IsADirError(Exception):
pass
class FileNotFoundError(Exception):
pass
class VFS(object):
def __init__(self):
self.root = dict()
def add_file(self, abspath, lookup=None, mkdirs=False):
names = self._split_path(abspath)
direc = self._mkdirp(names[:-1], srcpath=abspath, mkdirs=mkdirs)
filename = names[-1]
if filename in direc:
if isinstance(direc[filename], dict):
raise IsADirError(abspath)
return
direc[filename] = lookup
def lookup_file(self, abspath):
names = self._split_path(abspath)
direc = self._mkdirp(names[:-1], srcpath=abspath)
filename = names[-1]
if filename not in direc:
raise FileNotFoundError(Exception)
return direc[filename]
def _mkdirp(self, names, srcpath=None, mkdirs=False):
cur = self.root
for name in names:
if name not in cur:
if not mkdirs:
raise FileNotFoundError(srcpath)
cur[name] = dict()
elif not isinstance(cur, dict):
raise NotADirError(srcpath)
cur = cur[name]
return cur
def _split_path(self, path):
return path.strip(os.sep).split(os.sep)
<file_sep>/starfuse/pak/SBON.py
import collections
import struct
# Override range with xrange when running Python 2.x.
try:
range = xrange
except:
pass
Document = collections.namedtuple('Document', ['name', 'version', 'data'])
Tile = collections.namedtuple('Tile', [
'foreground_material',
'foreground_hue_shift',
'foreground_variant',
'foreground_sprite',
'foreground_sprite_hue_shift',
'background_material',
'background_hue_shift',
'background_variant',
'background_sprite',
'background_sprite_hue_shift',
'liquid',
'liquid_amount',
'liquid_pressure',
'liquid_unknown',
'collision',
'dungeon',
'biome',
'biome_2',
'indestructible',
])
def read_bytes(stream):
length = read_varlen_number(stream)
return stream.read(length)
def read_document(stream, repair=False):
name = read_string(stream)
# Not sure what this part is.
assert stream.read(1) == b'\x01'
version = struct.unpack('>i', stream.read(4))[0]
data = read_dynamic(stream, repair)
return Document(name, version, data)
def read_document_list(stream):
length = read_varlen_number(stream)
return [read_document(stream) for _ in range(length)]
def read_dynamic(stream, repair=False):
type = ord(stream.read(1))
try:
if type == 1:
return None
elif type == 2:
format = '>d'
elif type == 3:
format = '?'
elif type == 4:
return read_varlen_number_signed(stream)
elif type == 5:
return read_string(stream)
elif type == 6:
return read_list(stream, repair)
elif type == 7:
return read_map(stream, repair)
else:
raise ValueError('Unknown dynamic type 0x%02X' % type)
except:
if repair:
return None
raise
# Anything that passes through is assumed to have set a format to unpack.
return struct.unpack(format, stream.read(struct.calcsize(format)))[0]
def read_fixlen_string(stream, length):
return stream.read(length).rstrip(b'\x00').decode('utf-8')
def read_list(stream, repair=False):
length = read_varlen_number(stream)
return [read_dynamic(stream, repair) for _ in range(length)]
def read_map(stream, repair=False):
length = read_varlen_number(stream)
value = dict()
for _ in range(length):
key = read_string(stream)
value[key] = read_dynamic(stream, repair)
return value
def read_string(stream):
return read_bytes(stream).decode('utf-8')
def read_string_list(stream):
"""Optimized structure that doesn't have a type byte for every item.
"""
length = read_varlen_number(stream)
return [read_string(stream) for _ in range(length)]
def read_string_digest_map(stream):
"""Special structure of string/digest pairs, used by the assets database.
"""
length = read_varlen_number(stream)
value = dict()
for _ in range(length):
path = read_string(stream)
# Unnecessary whitespace.
stream.seek(1, 1)
digest = stream.read(32)
value[path] = digest
return value
def read_tile(world_version, stream):
if world_version < 6:
values = struct.unpack('>hBBhBhBBhBBHBhBB?', stream.read(23))
values = values[:12] + (0, 0) + values[12:]
else:
# TODO: Figure out what the new values mean.
values = struct.unpack('>hBBhBhBBhBBffBBhBB?', stream.read(30))
return Tile(*values)
def read_varlen_number(stream):
"""Read while the most significant bit is set, then put the 7 least
significant bits of all read bytes together to create a number.
"""
value = 0
while True:
byte = ord(stream.read(1))
if not byte & 0b10000000:
return value << 7 | byte
value = value << 7 | (byte & 0b01111111)
def read_varlen_number_signed(stream):
value = read_varlen_number(stream)
# Least significant bit represents the sign.
if value & 1:
return -(value >> 1)
else:
return value >> 1
def write_bytes(stream, bytes):
write_varlen_number(stream, len(bytes))
stream.write(bytes)
def write_varlen_number(stream, value):
if value == 0:
stream.write(b'\x00')
return
pieces = []
while value:
x, value = value & 0b01111111, value >> 7
if len(pieces):
x |= 0b10000000
pieces.insert(0, x)
if len(pieces) > 4096:
raise ValueError('Number too large')
stream.write(struct.pack('%dB' % len(pieces), *pieces))
def write_varlen_number_signed(stream, value):
has_sign = 1 if value < 0 else 0
write_varlen_number(stream, value << 1 | has_sign)
<file_sep>/starfuse/fs/mapped_file.py
"""A pseudo-file mapped into memory
Provides better performance for frequent reads/writes,
and makes reading/writing easier via regions (windows)
of memory. Allows memory to be accessed via array reads/
writes as well.
"""
import mmap
import logging
log = logging.getLogger(__name__)
class ReadOnlyError(Exception):
"""The mapped file is flagged as read-only"""
def __init__(self, path):
super(ReadOnlyError, self).__init__('mapped file is flagged as read only: %s' % path)
class RegionOverflowError(Exception):
"""Data at an offset was requested but the offset was greater than the allocated size"""
def __init__(self, offset):
super(RegionOverflowError, self).__init__('region overflow offset: %d (did you allocate?)' % offset)
class Region(object):
"""A virtual region of mapped memory
This class is a 'faked' mmap() result that allows for the finer allocation of memory mappings
beyond/below what the filesystem really allows. It is backed by true mmap()'d pages and
uses magic methods to achieve the appearance of being an isolated region of memory."""
__slots__ = 'parent', 'base_offset', '__size', 'cursor'
def __init__(self, parent, base_offset, size):
self.parent = parent
self.base_offset = base_offset
self.__size = size
self.cursor = 0
def __len__(self):
return self.__size
def __str__(self):
return str(self.read(offset=0, length=len(self)))
def __enter__(self):
return self
def __exit__(self, tipo, value, traceback):
return self
def region(self, offset=-1, size=-1):
(offset, size) = self._sanitize_segment(offset, size)
return self.parent.region(self.base_offset + offset, size)
def _sanitize_segment(self, offset, length):
if offset >= len(self):
raise ValueError('offset falls outside region size')
elif offset < 0:
offset = self.cursor
if length == 0:
raise ValueError('length must be at least 1')
elif length < 0:
length = len(self) - offset
return (offset, length)
def read(self, length=-1, offset=-1, advance=True):
(offset, length) = self._sanitize_segment(offset, length)
offset += self.base_offset
result = self.parent.read(length, offset, advance=advance)
if advance:
self.cursor += len(result)
return result
def write(self, value, length=-1, offset=-1, advance=True):
if length < 0:
length = len(value)
(offset, length) = self._sanitaize_segment(offset, length)
offset += self.base_offset
result = self.parent.write(value, length, offset, advance=advance)
if advance:
self.cursor += result
return result
class MappedFile(Region):
"""Manages mmap()-ings of a file into vmem.
This class prevents virtual address space from growing too large by
re-using existing maps if the requested regions have already been mapped.
"""
def __init__(self, path, page_count, read_only=False):
# XXX TODO NOTE remove this line when write functionality is added.
read_only = True
# getting 'too many files open' error? increase the constant on the next line
# (must be an exponent of 2)
self._page_size = page_count * mmap.PAGESIZE
# make sure we're sane here - allocation granularity needs to divide into page size!
assert (self._page_size % mmap.ALLOCATIONGRANULARITY) == 0, 'page size is not a multiple of allocation granularity!'
self._file = open(path, 'r+b')
self._pages = dict()
self.read_only = read_only
self._path = path
self.cursor = 0
super(MappedFile, self).__init__(self, base_offset=0, size=len(self))
def __len__(self):
self._file.seek(0, 2)
size = self._file.tell()
return size
def __del__(self):
self.close()
def close(self):
"""Unmaps all mappings"""
for i in self._pages:
self._pages[i].close()
self._file.close()
def region(self, offset, size):
"""Requests a virtual region be 'allocated'"""
lower_page = offset - (offset % self._page_size)
upper_page = ((offset + size) // self._page_size) * self._page_size
lower_page_id = lower_page // self._page_size
upper_page_id = upper_page // self._page_size
# make sure we're mapped
for i in range(lower_page_id, upper_page_id + 1):
if i not in self._pages:
page_offset = i * self._page_size
page_size = min(self._page_size, len(self) - page_offset)
log.debug('mapping vfile page: id=%d offset=%d size=%d', i, page_offset, page_size)
self._pages[i] = mmap.mmap(self._file.fileno(), offset=page_offset, length=page_size)
# create a region
return Region(self, base_offset=offset, size=size)
def read(self, length=1, offset=-1, advance=True):
"""Reads data from the virtual region"""
(offset, length) = self._sanitize_segment(offset, length)
results = []
length = min(length, len(self))
abs_offset = offset
cur_page = abs_offset // self._page_size
abs_offset %= self._page_size
while length > 0:
readable = self._page_size - abs_offset
readable = min(readable, length)
results.append(self._pages[cur_page][abs_offset:abs_offset + readable])
length -= readable
abs_offset = 0
cur_page += 1
result = ''.join(results)
if advance:
self.cursor += len(result)
return result
def write(self, value, offset=-1, length=-1, advance=True):
if self.read_only:
raise ReadOnlyError(self._path)
# TODO
assert False, 'not implemented'
return 0
def __getitem__(self, offset):
if isinstance(offset, slice):
(start, fin, step) = offset.indices(len(self))
result = self.read(offset=start, length=fin - start)
if step not in [None, 1]:
result = result[::step]
return result
if not isinstance(offset, int):
raise TypeError('offset is not an integer: %s' % repr(offset))
if offset >= len(self):
raise RegionOverflowError(offset)
page = offset // self._page_size
rel_offset = offset % self._page_size
return self._pages[page][rel_offset]
def __setitem__(self, offset, value):
if self.read_only:
raise ReadOnlyError(self._path)
if isinstance(offset, slice):
raise ValueError('Slice assignment not supported in mapped files; assemble your data first and then write')
if not isinstance(offset, int):
raise TypeError('offset is not an integer: %s' % repr(offset))
if offset >= len(self):
raise RegionOverflowError(offset)
page = offset // self._page_size
rel_offset = offset % self._page_size
self._pages[page][rel_offset] = value
<file_sep>/starfuse/fusepak.py
"""FUSE glue between the filesystem handlers and the PakVFS"""
import os
import errno
import logging
from threading import Lock
from fuse import FuseOSError, Operations, LoggingMixIn
from stat import S_IFDIR, S_IFREG
from time import time
from starfuse.pak.pakfile import Pakfile
from starfuse.fs.vfs import FileNotFoundError, IsADirError, NotADirError
log = logging.getLogger(__name__)
def fuse_op(fn):
def handled_fn(*args, **kwargs):
try:
return fn(*args, **kwargs)
except FileNotFoundError as e:
raise FuseOSError(errno.ENOENT)
except IsADirError as e:
raise FuseOSError(errno.EISDIR)
except NotADirError as e:
raise FuseOSError(errno.ENOTDIR)
except Exception as e:
log.exception('EIO: %s', e.message)
raise FuseOSError(errno.EIO)
return handled_fn
class FusePAK(LoggingMixIn, Operations):
"""FUSE operations implementation for StarBound PAK files"""
def __init__(self, pakfile, page_count, read_only=False):
self.pakfile = Pakfile(pakfile, page_count, read_only=read_only)
self._lock = Lock()
def make_file_struct(self, size, isfile=True, ctime=time(), mtime=time(), atime=time(), read_only=False):
stats = dict()
# TODO replace uncommented modes with commented when write ability is added
if isfile:
stats['st_mode'] = S_IFREG | 0o0444
else:
stats['st_mode'] = S_IFDIR | 0o0555
if not self.pakfile.read_only:
stats['st_mode'] |= 0o0200
stats['st_uid'] = os.getuid()
stats['st_gid'] = os.getgid()
stats['st_nlink'] = 1
stats['st_ctime'] = ctime
stats['st_mtime'] = mtime
stats['st_atime'] = atime
stats['st_size'] = size
return stats
@fuse_op
def access(self, path, mode):
return 0
@fuse_op
def flush(self, path, fn):
return 0
@fuse_op
def fsync(self, path, datasync, fh):
# we don't need to worry about fsync in StarFuse. :)
return 0
@fuse_op
def getattr(self, path, fh=None):
if path == '/':
return self.make_file_struct(0, isfile=False)
(_, _, _, isfile) = self.pakfile.entry(path)
if not isfile:
return self.make_file_struct(0, isfile=False)
size = self.pakfile.file_size(path)
return self.make_file_struct(size)
@fuse_op
def readdir(self, path, fh=None):
return self.pakfile.directory_listing(path)
@fuse_op
def read(self, path, size, offset, fh=None):
return self.pakfile.file_contents(path, offset, size)
| a685326d70983a6d4e89137fc94f7238cf925ccc | [
"Markdown",
"Python"
] | 11 | Python | Qix-/starfuse | 91602ae6b6553a113a26418bf628f29be867ee78 | 79dfdc7b7b8f12fb7eedb39fe223283b5f3bede0 | |
refs/heads/master | <repo_name>CHaram0913/twitch-project-react<file_sep>/models/LogSchema.js
let mongoose = require('mongoose');
let LogSchema = mongoose.Schema({
log_time: {
type: Number,
required: true
},
user_id: {
type: String,
required: true
},
user_name: {
type: String,
required: true
},
viewer_count: {
type: Number,
required: true
},
follower_count: {
type: Number,
required: true
},
stream_title: {
type: String,
required: true
},
start_time: {
type: Number,
required: true
},
game_id: {
type: String,
required: true
},
game_name: {
type: String,
required: true
},
game_art: {
type: String,
required: true
}
});
module.exports = { LogSchema };<file_sep>/client/src/resource/colorArray.js
const generateRandomColor = () => {
let letters = '0123456789ABCEDF';
let color = '#';
for (let i = 0; i < 6; i++) {
color += letters[Math.floor(Math.random() * 16)];
}
return color;
};
const findMatch = (colorArray, key) => {
return colorArray.find(function(element) {
return element.game === key;
})
};
export const setColor = (gameArray, colorTheme) => {
let colorArray = [];
for (let i = 0; i < gameArray.length; i++) {
let matchFound = findMatch(colorTheme, gameArray[i]);
if (matchFound) {
colorArray.push(matchFound.color);
} else {
colorArray.push(generateRandomColor());
}
}
return colorArray;
};
export const colorSet = [
{
game : 'League of Legends',
color : '#FFCE56'
},
{
game : 'Hearthstone',
color : '#36A2EB'
},
{
game : 'IRL',
color : '#FF6384'
},
{
game : 'Games + Demos',
color : '#840396'
},
{
game : 'FIFA 18',
color : '#FCF2FC'
},
{
game : 'Stardew Valley',
color : '#66FFB3'
},
{
game : 'Overwatch',
color : '#BCBABE'
},
{
game : 'Heavy Rain',
color : '#323233'
},
{
game : "PLAYERUNKNOWN'S BATTLEGROUNDS",
color : '#124F2C'
},
{
game : "Mount & Blade: Warband",
color : '#BBBB77'
},
{
game : "Celeste",
color : '#99FFFF'
},
{
game : "StarCraft II",
color : '#FF3333'
},
{
game : "Princess Maker 5",
color : '#33FFCC'
},
{
game : "MapleStory",
color : '#FF99FF'
},
{
game : "Cookie Run: OvenBreak",
color : '#86592D'
},
{
game : "Slay the Spire",
color : '#E65C00'
},
{
game : "Creative",
color : '#FFFF80'
},
];<file_sep>/query_helper/filter_stream_by_length.js
const filterStreamByLength = (streamsArray) => {
let longEnoughStream = [];
for (let i = 0; i < streamsArray.length; i++) {
let fullCount = streamsArray[i].map(stream => stream.count).reduce((acc, val) => acc + val);
if (fullCount > 14) {
longEnoughStream.push(streamsArray[i]);
}
}
return longEnoughStream;
};
module.exports = { filterStreamByLength };<file_sep>/client/src/components/gamelist_pie_viewer.js
import _ from 'lodash';
import { colorSet, setColor } from './../resource/colorArray';
export default function(props) {
const colorArray = setColor(_.map(props, '_id'), colorSet);
const data = {
labels: _.map(props, '_id'),
datasets: [{
data: _.map(props, 'average_view'),
backgroundColor: colorArray,
hoverBackgroundColor: colorArray
}]
}
return data;
};<file_sep>/client/src/containers/stream_stat.js
import React, { Component, Fragment } from 'react';
import { connect } from 'react-redux';
import { Line, HorizontalBar } from 'react-chartjs-2';
import moment from 'moment-timezone';
import _ from 'lodash';
import { withStyles } from 'material-ui/styles';
import { Paper, Typography, Grid, Hidden } from 'material-ui';
import streamStatStyles from './../styles/stream_stat_styles';
import { fetchStreamStat } from './../actions/index';
import { barData, lineData } from './../components/stream_graphs';
import BarChartOption from './../styles/bar_chart_options';
import LineChartOption from './../styles/line_chart_options';
class StreamStat extends Component {
componentDidUpdate(prevProps) {
if ((prevProps.streamerName === this.props.streamerName) && (prevProps.mode === this.props.mode)) {
} else {
this.props.fetchStreamStat(this.props.streamerName, this.props.mode);
}
}
convertCount(singleStream) {
let min = singleStream.map(stream => stream.count).reduce((acc, val) => acc + val) * 10 ;
let hours = Math.floor(min / 60);
let minutes = min % 60;
if (hours === 0) {
return `${minutes} minutes`;
} else {
return `${hours} hours ${minutes} minutes`;
}
}
averageView(singleStream) {
let viewerArray = [];
for (let i = 0; i < singleStream.length; i++) {
for (let j = 0; j < singleStream[i].at_time.length; j++) {
viewerArray.push(singleStream[i].at_time[j].viewer_count);
}
}
let averageViewer = Math.floor(viewerArray.reduce((acc, val) => acc + val) / viewerArray.length) ;
return averageViewer;
}
followDifference(singleStream) {
let follower_at_start = singleStream[0].at_time[0].follower_count ;
let follower_at_end = singleStream[singleStream.length - 1].at_time[singleStream[singleStream.length - 1].at_time.length - 1].follower_count ;
return follower_at_end - follower_at_start ;
}
render() {
const { streamlist } = this.props;
if (!streamlist.data) {
return <div>Fetching streamlist...</div>;
}
const streamStatGraph = (streamArray) => {
const { classes } = this.props;
let graphArray = [];
for (let i = 0; i < streamArray.length; i++){
const generate_game_list = (singleStream) => {
return _.uniq(singleStream.map(stream => stream._id.game_name));
};
graphArray.push (
<Paper className={classes.root} elevation={5} key={i}>
<Grid container spacing={8} className={classes.container} direction='row-reverse' alignItems='center'>
<Grid item xs={12} zeroMinWidth>
<Paper className={classes.title} elevation={2}>
<Typography variant='title' className={classes.title_text}>
{`Stream at: ${moment(streamArray[i][0].start_time).tz('Asia/Seoul').format('(YYYY MMM DD) hh:mm a')}`}
</Typography>
</Paper>
</Grid>
<Grid item xs={12} md={2} zeroMinWidth >
<Paper className={classes.detail} elevation={0}>
<Typography className={classes.detail_title}>
Stream Stat
</Typography>
<Typography className={classes.detail_subtitle}>
Games Played:
</Typography>
{generate_game_list(streamArray[i]).map(game =>
<Typography className={classes.detail_component} key={game}>
{game}
</Typography>
)}
<Typography className={classes.detail_subtitle}>
Stream Length:
</Typography>
<Typography className={classes.detail_component}>
{this.convertCount(streamArray[i])}
</Typography>
<Typography className={classes.detail_subtitle}>
Average Viewers:
</Typography>
<Typography className={classes.detail_component}>
{`${this.averageView(streamArray[i])} viewers`}
</Typography>
<Typography className={classes.detail_subtitle}>
Followers Gained:
</Typography>
<Typography className={classes.detail_component}>
{`${this.followDifference(streamArray[i])} more followers!`}
</Typography>
</Paper>
</Grid>
<Hidden xsDown>
<Grid item xs={12} md={10} zeroMinWidth>
<Paper className={classes.bar} elevation={0}>
<HorizontalBar data={barData(streamArray[i])} options={BarChartOption(streamArray[i])} />
</Paper>
<Paper className={classes.line} elevation={0}>
<Line data={lineData(streamArray[i])} height={300} options={LineChartOption} />
</Paper>
</Grid>
</Hidden>
</Grid>
</Paper>
)
}
return graphArray;
};
return (
<Fragment>
{streamStatGraph(streamlist.data)}
</Fragment>
);
}
};
function mapStateToProps(state) {
return {
streamerName : state.streamerName,
mode : state.mode,
streamlist : state.streamlist
}
}
export default withStyles (streamStatStyles) (connect (mapStateToProps, { fetchStreamStat }) (StreamStat));<file_sep>/client/src/reducers/index.js
import { combineReducers } from 'redux';
import SelectedStreamerReducer from './reducer_selected_stream';
import SelectedModeReducer from './reducer_selected_mode';
import GameListReducer from './reducer_game_list';
import StreamListReducer from './reducer_stream_list';
import StreamStatus from './reducer_stream_status';
const rootReducer = combineReducers({
streamerName : SelectedStreamerReducer,
mode : SelectedModeReducer,
gamelist : GameListReducer,
streamlist : StreamListReducer,
status: StreamStatus
});
export default rootReducer;<file_sep>/client/src/actions/index.js
import axios from 'axios';
import { streamerArray } from './../resource/streamerArray';
import { STREAMER_SELECTED, MODE_SELECTED, FETCH_GAME_STAT, FETCH_STREAM, FETCH_STREAM_STATUS } from './types';
export function streamSelect(streamerIndex) {
return {
type: STREAMER_SELECTED,
payload: streamerArray[streamerIndex]
};
};
export function modeSelect(mode) {
return {
type: MODE_SELECTED,
payload: mode
};
};
export function fetchGameStat(collectionName, mode) {
return async dispatch => {
let response = await axios.get(`/api/gamelist/${collectionName}/${mode}`);
dispatch({
type : FETCH_GAME_STAT,
payload : response
});
};
};
export function fetchStreamStat(collectionName, mode) {
return async dispatch => {
let response = await axios.get(`/api/allStream/${collectionName}/${mode}`);
dispatch({
type : FETCH_STREAM,
payload : response
});
};
};
export function fetchStreamStatus(collectionName) {
return async dispatch => {
let response = await axios.get(`/api/status/${collectionName}`);
dispatch({
type : FETCH_STREAM_STATUS,
payload : response
});
};
};
<file_sep>/client/src/containers/game_stat.js
import React, { Component } from 'react';
import { connect } from 'react-redux';
import { Doughnut } from 'react-chartjs-2';
import _ from 'lodash';
import { withStyles } from 'material-ui/styles';
import { Paper, Grid, GridList, GridListTile, GridListTileBar, Hidden } from 'material-ui';
import gameStatStyles from './../styles/game_stat_styles';
import { fetchGameStat } from './../actions/index';
import PieStreamTime from './../components/gamelist_pie_time';
import PieAverageView from './../components/gamelist_pie_viewer';
import { pie_time_option, pie_view_option } from './../styles/pie_chart_options';
import GameData from './../components/gamelist_image_data';
class GameStat extends Component {
componentDidUpdate(prevProps) {
if ((prevProps.streamerName === this.props.streamerName) && (prevProps.mode === this.props.mode)) {
} else {
this.props.fetchGameStat(this.props.streamerName, this.props.mode);
}
}
render() {
const { gamelist } = this.props;
const { classes } = this.props;
if (!gamelist.data) {
return <div>Fetching gamelist...</div>;
}
let game_art_data = GameData((_.sortBy(gamelist.data, 'count')).reverse());
return (
<Grid container spacing={8} className={classes.root} direction='row-reverse' alignItems='center'>
<Grid item xs={12} md={12} lg={5} zeroMinWidth>
<Paper className={classes.gamelist} elevation={4}>
<GridList cellHeight={200} cols={4} className={classes.gridlist}>
{game_art_data.map(game =>
<GridListTile key={game.title}>
<img src={game.img} alt={game.title} />
<GridListTileBar
title={game.title}
// subtitle={`Time: ${game.duration} \n View: ${game.viewer}`}
className={classes.tile_bar}
/>
</GridListTile>
)}
</GridList>
</Paper>
</Grid>
<Hidden xsDown>
<Grid item xs={12} md={6} lg={true} zeroMinWidth>
<Paper className={classes.pie} elevation={4}>
<Doughnut data={PieStreamTime(gamelist.data)} options={pie_time_option}/>
</Paper>
</Grid>
<Grid item xs={12} md={6} lg={true} zeroMinWidth>
<Paper className={classes.pie} elevation={4}>
<Doughnut data={PieAverageView(gamelist.data)} options={pie_view_option}/>
</Paper>
</Grid>
</Hidden>
</Grid>
);
}
};
function mapStateToProps(state) {
return {
streamerName : state.streamerName,
mode : state.mode,
gamelist : state.gamelist
}
}
export default withStyles (gameStatStyles) (connect (mapStateToProps, { fetchGameStat }) (GameStat));<file_sep>/client/src/styles/bar_chart_options.js
export default function (props) {
let max = props.map(count => count.count).reduce((acc, val) => acc + val);
return {
maintainAspectRatio: false,
legend: {
display: false
},
layout: {
padding: {
left: 0,
right: 0,
top: 0,
bottom: 0
}
},
scales: {
xAxes: [{
stacked: true,
display: false,
ticks: {
beginAtZero: true,
maxTicksLimit: 2,
max: max
}
}],
yAxes: [{
stacked: true,
display: false,
maxBarThickness: 70
}]
},
tooltips: {
mode: 'point',
position: 'nearest',
callbacks: {
title: function(t, d){
return d.datasets[t[0].datasetIndex].label[1];
},
label: function(t, d) {
let minuteTotal = d.datasets[t.datasetIndex].data[0] * 10;
let hours = Math.floor(minuteTotal / 60);
let minutes = minuteTotal % 60;
if (hours === 0) {
return `${d.datasets[t.datasetIndex].label[0]}: ${minutes}min`;
} else {
return `${d.datasets[t.datasetIndex].label[0]}: ${hours}hr ${minutes}min`;
}
}
}
}}
};<file_sep>/aggregates/stream_aggregate.js
const each_stream_aggregate = (start_time) => {
return [
{
$match : { 'start_time' : { $in : start_time } }
},
{
$group : {
_id : { game_name : '$game_name', stream_title : '$stream_title'},
count : { $sum : 1 },
game_art : { $first : '$game_art'},
at_time : { $push : { log_time : '$log_time', viewer_count : '$viewer_count', follower_count : '$follower_count' } },
start_game_time : { $first : '$log_time' }
}
},
{
$sort : { start_game_time : 1 }
},
// {
// $group : {
// _id : '$_id.game_name',
// title: { $first : '$_id.stream_title' },
// count : { $sum : 1 },
// game_art : { $first : '$game_art'},
// }
// }
// {
// $group : {
// _id : '$game_name',
// count : { $sum : 1 },
// at_time : { $push : { log_time : '$log_time', viewer_count : '$viewer_count', follower_count : '$follower_count' } },
// game_art : { $first : '$game_art'},
// start_game_time : { $first : '$log_time' }
// }
// }
// {
// $sort : { start_game_time : 1 }
// },
{
$project : {
count : '$count',
start_time : '$start_game_time',
game_art : '$game_art',
at_time : '$at_time'
}
}
];
}
module.exports = { each_stream_aggregate };<file_sep>/client/src/actions/types.js
export const STREAMER_SELECTED = 'streamer_selected';
export const MODE_SELECTED = 'mode_selected';
export const FETCH_GAME_STAT = 'fetch_game_stat';
export const FETCH_STREAM = 'fetch_stream';
export const FETCH_STREAM_STATUS = 'fetch_stream_status';<file_sep>/client/src/containers/remote_control.js
import React, { Component, Fragment } from 'react';
import { connect } from 'react-redux';
import { animateScroll as scroll } from 'react-scroll';
import { withStyles } from 'material-ui/styles';
import remoteControlStyles from './../styles/remote_control_styles';
import { Paper, Popover, Button } from 'material-ui';
import Radio, { RadioGroup } from 'material-ui/Radio';
import { FormLabel, FormControl, FormControlLabel } from 'material-ui/Form';
import RemoteIcon from '@material-ui/icons/Timelapse';
import UpIcon from '@material-ui/icons/ArrowUpward';
import periodArray from './../resource/periodArray';
import { modeSelect, fetchStreamStatus } from './../actions/index';
class RemoteControl extends Component {
constructor(props) {
super(props);
this.state = {
check : 'month',
anchorEl : null
}
this.onSelection = this.onSelection.bind(this);
this.openPopover = this.openPopover.bind(this);
this.closePopover = this.closePopover.bind(this);
this.createStatusButton = this.createStatusButton.bind(this);
}
onSelection(event, value) {
this.props.modeSelect(value);
this.setState({ check : value });
}
componentDidUpdate(prevProps) {
if ((prevProps.streamerName === this.props.streamerName) && (prevProps.mode === this.props.mode)) {
} else {
this.props.fetchStreamStatus(this.props.streamerName);
}
}
componentDidMount() {
this.props.modeSelect(this.state.check);
}
openPopover(event) {
this.setState({
anchorEl : event.currentTarget
});
}
closePopover(event) {
this.setState({
anchorEl : null
});
}
scrollToTop() {
scroll.scrollToTop();
}
createStatusButton(status) {
try {
if (status.data.status === 'LIVE') {
return (
<Button
variant="raised"
href={`https://www.twitch.tv/${this.props.streamerName}`}
target='_blank'
className={this.props.classes.live_link_online}
>
LIVE
</Button>
)
} else {
return (
<Button
variant="raised"
className={this.props.classes.live_link_offline}
>
OFFLINE
</Button>
)
}
} catch (e) {
return (
<div />
)
}
}
render() {
const { classes } = this.props;
return (
<Fragment>
<Button
className={classes.scrollTopButton}
variant='fab'
color='secondary'
onClick={this.scrollToTop}
>
<UpIcon />
</Button>
<Button
className={classes.remoteButton}
variant='fab'
color='primary'
onClick={this.openPopover}
>
<RemoteIcon />
</Button>
<Popover
open={Boolean(this.state.anchorEl)}
anchorEl={this.state.anchorEl}
onClose={this.closePopover}
anchorOrigin={{
vertical: 'center',
horizontal: 'center'
}}
transformOrigin={{
vertical: 'bottom',
horizontal: 'right'
}}
>
<Paper className={classes.remotePaper}>
<FormControl component='fieldset' required className={classes.formControl}>
<FormLabel component='legend'>Time Period</FormLabel>
<RadioGroup
aria-label='time'
name='search_period_selection'
className={classes.group}
value={this.state.check}
onChange={this.onSelection}
>
{periodArray.map(time_periods =>
<FormControlLabel
key={time_periods}
value={time_periods}
control={<Radio />}
label={time_periods}
/>
)}
</RadioGroup>
{this.createStatusButton(this.props.status)}
</FormControl>
</Paper>
</Popover>
</Fragment>
);
};
}
function mapStateToProps(state) {
return {
streamerName : state.streamerName,
mode : state.mode,
status : state.status
}
}
export default withStyles (remoteControlStyles) (connect (mapStateToProps, { modeSelect, fetchStreamStatus }) (RemoteControl));<file_sep>/query_helper/slice_stream_aggregate.js
const _ = require('lodash');
function createArray(length) {
var arr = new Array(length || 0),
i = length;
if (arguments.length > 1) {
var args = Array.prototype.slice.call(arguments, 1);
while (i--) arr[length -1 - i] = createArray.apply(this, args);
}
return arr;
}
const slice_aggregate = (singleStream) => {
let jump = createArray(singleStream.length, 0);
for (let i = 0; i < singleStream.length; i++) {
let prev = 0;
for (let j = 0; j < singleStream[i].at_time.length; j++) {
if (singleStream[i].at_time[j].log_time - prev > 50 * 60 * 1000) {
prev = singleStream[i].at_time[j].log_time;
if (j !== 0) {
jump[i].push({i, j})
}
} else {
prev = singleStream[i].at_time[j].log_time;
}
}
}
const separate_at_time = (stream_array, jumpArray) => {
let at_time_one = stream_array.at_time;
let newArray = createArray(jumpArray.length + 1, 0);
let j = 0;
for (let i = 0; i < at_time_one.length; i++) {
if (i === 0) {
newArray[j].push(at_time_one[i]);
} else {
if (j < jumpArray.length) {
if (at_time_one[i].log_time < at_time_one[jumpArray[j].j].log_time) {
newArray[j].push(at_time_one[i]);
} else if (at_time_one[i].log_time === at_time_one[jumpArray[j].j].log_time){
j++ ;
newArray[j].push(at_time_one[i]);
}
} else if (j === jumpArray.length) {
newArray[j].push(at_time_one[i]);
}
}
}
return newArray;
}
let finalArray = [];
let finalFinal = [];
let sortJump = jump.filter(function(sub) {
return sub.length;
});
if (sortJump.length > 0) {
for (let i = 0; i < singleStream.length; i++) {
if (jump[i]) {
finalArray.push(separate_at_time(singleStream[i], jump[i]));
} else {
finalArray.push(singleStream[i]);
}
}
for (let i = 0; i < finalArray.length; i++) {
for (let j = 0; j < finalArray[i].length; j++) {
finalFinal.push({
_id: {
game_name: singleStream[i]._id.game_name,
stream_title: singleStream[i]._id.stream_title
},
count: finalArray[i][j].length,
start_time: finalArray[i][j][0].log_time,
game_art: singleStream[i].game_art,
at_time: finalArray[i][j]
})
}
}
return (_.sortBy(finalFinal, 'start_time'));
} else {
return singleStream;
}
};
module.exports = { slice_aggregate };<file_sep>/client/src/styles/line_chart_options.js
import moment from 'moment-timezone';
export default {
maintainAspectRatio: false,
legend: {
display: true,
width: '12px',
labels: {
fontColor: 'rgba(252, 255, 255, 0.9)',
fontSize: 14
},
position: 'bottom'
},
layout: {
padding: {
left: 0,
right: 0,
top: 0,
bottom: 0
}
},
elements: {
point: {
pointStyle: 'star',
radius: 5
},
line: {
fill: false,
tension: 0.2,
borderCapStyle: 'round',
}
},
scales: {
border: '1px',
yAxes: [{
id: 'Views',
position: 'left',
display: true,
gridLines: {
display: false,
color: 'rgba(252, 255, 255, 0.9)',
lineWidth: 2,
drawTicks: false,
zeroLineWidth: 2,
zeroLineColor: 'rgba(252, 255, 255, 0.9)'
},
ticks: {display: false}
}, {
id: 'Follows',
position: 'right',
display: true,
gridLines: {
display: false,
color: 'rgba(252, 255, 255, 0.9)',
lineWidth: 2,
drawTicks: false,
zeroLineWidth: 2,
zeroLineColor: 'rgba(252, 255, 255, 0.9)'
},
ticks: {display: false}
}],
xAxes: [{
position: 'bottom',
gridLines: {
display: false,
color: 'rgba(252, 255, 255, 0.9)',
lineWidth: 2,
drawTicks: false,
zeroLineWidth: 2,
zeroLineColor: 'rgba(252, 255, 255, 0.9)'
},
type: 'time',
time: {
unit: 'hour',
millisecond: 'h:mm a'
},
ticks: {
callback: function(t) {
return t;
},
fontColor: 'rgba(252, 255, 255, 0.9)',
fontSize: 14,
maxRotation: 0,
minRotation: 0,
padding: 5
}
}]
},
tooltips: {
callbacks: {
title: function(t, d) {
return moment(d.labels[t[0].index]).tz('Asia/Seoul').format('YYYY MMM DD, hh:mm:ss a') ;
}
},
mode: 'index',
position: 'nearest'
}
};<file_sep>/client/src/styles/top_menu_styles.js
export default
{
root: {
flexGrow: 1,
},
tabs: {
background: 'linear-gradient(180deg, #8c02cc 30%, #c650fc 90%)',
border: 0,
color: 'white',
boxShadow: '0 3px 5px 2px rgba(255, 105, 135, .30)',
},
tab: {
fontFamily: 'Roboto, sans-serif',
},
};<file_sep>/client/src/styles/stream_stat_styles.js
export default
{
root: {
height: 'auto',
backgroundColor: 'rgba(40, 44, 52, 1)',
flexGrow: 1,
marginTop: 25,
marginBottom: 25,
paddingTop: 10,
paddingBottom: 20
},
container: {
height: '100%',
},
title: {
height: 'auto',
backgroundColor: 'rgba(40, 44, 52, 1)'
},
title_text: {
color: 'white',
textAlign: 'center',
fontWeight: 'bold',
paddingBottom: 5
},
bar: {
backgroundColor: 'rgba(40, 44, 52, 1)',
paddingLeft: 18,
paddingRight: 18
},
line: {
backgroundColor: 'rgba(40, 44, 52, 1)'
},
detail: {
height: 'auto',
width: 230,
borderRadius: '13%',
backgroundColor: 'rgba(181, 71, 232, 0.7)',
paddingBottom: 15,
marginLeft: 'auto',
marginRight: 'auto'
},
detail_title: {
color: 'white',
textAlign: 'center',
fontSize: 30,
fontWeight: 'bold',
paddingTop: 10
},
detail_subtitle: {
color: 'white',
textAlign: 'left',
fontSize: 23,
fontWeight: 'bold',
fontStyle: 'oblique',
paddingTop: 8,
paddingBottom: 2,
paddingLeft: 18,
},
detail_component: {
color: 'white',
textAlign: 'left',
fontSize: 17,
paddingLeft: 28
}
};<file_sep>/client/src/routes/index.js
/**
* Root
* */
import RootRoute from './root/root';
import StreamerStat from './root/streamer_stat';
/**
* Exports
* */
const mainRoutes = [
{
path: '/',
component:RootRoute,
wrapper:''
},
{
path: '/streamers',
component:StreamerStat,
wrapper:''
}
];
export{
mainRoutes
}<file_sep>/client/src/routes/root/root.js
import React, { Component, Fragment } from 'react';
import { withStyles } from 'material-ui/styles';
// import Welcome from './../../components/welcome';
import GameStat from './../../containers/game_stat';
import StreamStat from './../../containers/stream_stat';
const styles = theme => ({
root: {}
});
class Root extends Component {
render() {
return (
<Fragment>
<GameStat />
<StreamStat />
</Fragment>
// <Welcome />
)
}
}
export default withStyles(styles)(Root);<file_sep>/client/src/resource/periodArray.js
export default ['all', 'month', 'week', 'day'];<file_sep>/query_helper/get_start_time_comparison.js
const moment = require('moment-timezone');
const getStartTimeComp = (mode) => {
if (mode === 'day') {
// Setting the base as Korea, today at 00:00:00
let currentDateKorea = moment().tz('Asia/Seoul').format('YYYYMMDD');
let dateKorea = `${currentDateKorea}T000000`;
let KoreaUTC = Number(moment(dateKorea).format('x'));
let dateUTC = KoreaUTC - 9 * 60 * 60 * 1000;
return dateUTC - 24 * 60 * 60 * 1000;
} else if (mode === 'week') {
let currentDateKorea = moment().tz('Asia/Seoul').format('YYYYMMDD');
let dateKorea = `${currentDateKorea}T000000`;
let KoreaUTC = Number(moment(dateKorea).format('x'));
let dateUTC = KoreaUTC - 9 * 60 * 60 * 1000;
return dateUTC - 7 * 24 * 60 * 60 * 1000;
} else if (mode === 'month') {
let currentDateKorea = moment().tz('Asia/Seoul').format('YYYYMMDD');
let dateKorea = `${currentDateKorea}T000000`;
let KoreaUTC = Number(moment(dateKorea).format('x'));
let dateUTC = KoreaUTC - 9 * 60 * 60 * 1000;
return dateUTC - 30 * 24 * 60 * 60 * 1000;
} else if (mode === 'all') {
return 0;
}
}
module.exports = { getStartTimeComp }; | 14ce7452591a873b2dcba9711580e2ed4f9cf5f7 | [
"JavaScript"
] | 20 | JavaScript | CHaram0913/twitch-project-react | 748f84f61c39cf5bac3ea7cb868cae8ab26ad510 | 3cd74966ca35e927246a12b63d8130535343c286 | |
refs/heads/master | <repo_name>Clemsx/Csharp<file_sep>/DOT_cardGames_2017/cardGame/cardGame/Hand.cs
using System.Collections.Generic;
namespace cardGame
{
public class Hand
{
private List<List<Card>> _hands;
private List<bool> _hasPlay;
public Hand()
{
_hands = new List<List<Card>>();
_hands.Add(new List<Card>());
_hasPlay = new List<bool>();
_hasPlay.Add(false);
}
public Card Card
{
get => default(Card);
set
{
}
}
public void SetHasPlay(int index, bool play) => _hasPlay[index] = play;
public bool GetHasPlay(int index) => _hasPlay[index];
public void ClearHand(int index = 0)
{
if (_hands[0].Count != 0)
_hands[index].Clear();
}
public void TakeCard(Card card, int index = 0) => _hands[index].Add(card);
public int GetHandValue(int index = 0)
{
int value = 0;
int cardValue;
foreach (var card in _hands[index])
{
cardValue = card.GetValue();
if (cardValue == 1)
value += (value + 11 > 21 ? 1 : 11);
else
value += cardValue;
}
return value;
}
public string GetDisplaySingleHandMsg(int index = 0, bool dealer = false)
{
string msg = "";
int value = GetHandValue();
bool first = true;
msg += value.ToString();
msg += " (";
foreach (var card in _hands[0])
{
if (first == false)
msg += " + ";
msg += card.GetCardString();
first = false;
}
msg += ")";
if (dealer)
return "Dealer's hand:\r\n\t" + msg;
return "Your hand:\r\n\t" + msg;
}
public string GetDisplaySplitHandMsg()
{
string msg = "";
bool first = true;
for (int i = 0; i < _hands.Count; i++)
{
if (first == false)
msg += "[Server]: ";
msg += GetDisplaySingleHandMsg(i);
first = false;
}
return msg;
}
public string GetDisplayHandMsg(int index = 0, bool dealer = false)
{
if (_hands.Count > 1)
return GetDisplaySplitHandMsg();
return GetDisplaySingleHandMsg(index, dealer);
}
public string GetDisplayHidHandMsg()
{
string msg = "";
int value = _hands[0][0].GetValue();
msg += value.ToString() +
" (" + _hands[0][0].GetCardString() + ")";
return "Dealer's hand:\r\n\t" + msg;
}
public bool IsBusted(int index = 0)
{
int value = GetHandValue(index);
if (value > 21)
return true;
else
return false;
}
public List<Card> GetHand(int index = 0)
{
if (index < _hands.Count)
return _hands[index];
return null;
}
public void SplitCard(int index = 0)
{
Card card = _hands[index][1];
_hands[index].RemoveAt(1);
_hands[index + 1] = new List<Card>();
_hands[index + 1].Add(card);
_hasPlay.Add(false);
}
public bool IsDoublable(int index)
{
if (_hands[index].Count == 2)
return true;
return false;
}
public bool IsSplittable(int index)
{
if (_hands[index][0].GetValue() == _hands[index][1].GetValue())
return true;
return false;
}
public string GetPlayerActionMsg()
{
string msg = "Would you like to HIT, STAND";
if (IsDoublable(0))
msg += ", DOUBLE";
msg += " ?";
return msg;
}
public bool IsEmpty()
{
if (_hands[0].Count == 0)
return true;
return false;
}
}
}<file_sep>/DOT_AREA_2017/DOT_AREA_2017/DOT_AREA_2017/Home/API/Twitter.aspx.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using mailinblue;
using Vianett.Common.Log4net;
using System.Net;
using System.IO;
using System.Security.Cryptography;
using System.Text;
using Facebook;
using Tweetinvi;
using System.Threading.Tasks;
using Dropbox.Api;
using Dropbox.Api.Files;
using DOT_AREA_2017.Home.Others;
namespace DOT_AREA_2017
{
public partial class Twitter : Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
protected void ButtonMail_Click(object sender, EventArgs e)
{
TwitterPerso TP = new TwitterPerso();
string message = TextTwitter.InnerText;
string oauth_consumer_key = TP.getConsumerKey();
string oauth_consumer_secret = TP.getConsumerSecret();
string oauth_token = TP.getToken();
string oauth_token_secret = TP.getTokenSecret();
///////////////////////////////////////////////// SEND IN BLUE //////////////////////////////////////////////////
API sendinBlue = new mailinblue.API("ERWXqjT4mS3aA05V", 5000); //Optional parameter: Timeout in MS
Dictionary<string, Object> data = new Dictionary<string, Object>();
Dictionary<string, string> to = new Dictionary<string, string>();
to.Add(TextDestination.Text, "to whom!");
List<string> from_name = new List<string>();
from_name.Add("<EMAIL>");
from_name.Add("AREA.NET");
//List<string> attachment = new List<string>();
//attachment.Add("https://domain.com/path-to-file/filename1.pdf");
//attachment.Add("https://domain.com/path-to-file/filename2.jpg");
data.Add("to", to);
data.Add("from", from_name);
data.Add("subject", "AREA send in blue");
data.Add("html", String.Concat("You post the following message on Twitter : ", message));
//data.Add("attachment", attachment);
/////////////////////////////////////////////////////////////////////////////////////////////////////////////////
Auth.SetUserCredentials(oauth_consumer_key, oauth_consumer_secret, oauth_token, oauth_token_secret);
try
{
LabelResult.ForeColor = System.Drawing.Color.Green;
LabelResult.Visible = true;
LabelResult.Text = "Message posted on Twitter and sended to " + TextDestination.Text;
Object sendEmail = sendinBlue.send_email(data);
Tweet.PublishTweet(message);
}
catch (Exception ex)
{
LabelResult.ForeColor = System.Drawing.Color.Red;
LabelResult.Visible = true;
LabelResult.Text = ex.Message;
}
}
protected void ButtonPhone_Click(object sender, EventArgs e)
{
string message = TextTwitter.InnerText;
TwitterPerso TP = new TwitterPerso();
string oauth_consumer_key = TP.getConsumerKey();
string oauth_consumer_secret = TP.getConsumerSecret();
string oauth_token = TP.getToken();
string oauth_token_secret = TP.getTokenSecret();
Auth.SetUserCredentials(oauth_consumer_key, oauth_consumer_secret, oauth_token, oauth_token_secret);
///////////////////////////////////////////////// VIANETT //////////////////////////////////////////////////
string username = "<EMAIL>";
string password = "<PASSWORD>";
string msgsender = "33683821203";
string destinationaddr = string.Concat("33", TextDestination.Text.Remove(0, 1).ToString());
ViaNettSMS s = new ViaNettSMS(username, password);
ViaNettSMS.Result result;
/////////////////////////////////////////////////////////////////////////////////////////////////////////////////
try
{
Tweet.PublishTweet(message);
result = s.sendSMS(msgsender, destinationaddr, String.Concat("You post on Twitter : ", message));
LabelResult.ForeColor = System.Drawing.Color.Green;
LabelResult.Visible = true;
LabelResult.Text = "Message posted on Twitter and sended to " + TextDestination.Text + " !";
}
catch (Exception ex)
{
LabelResult.ForeColor = System.Drawing.Color.Red;
LabelResult.Visible = true;
LabelResult.Text = ex.Message;
}
}
protected void ButtonFb_Click(object sender, EventArgs e)
{
string message = TextTwitter.InnerText;
TwitterPerso TP = new TwitterPerso();
string oauth_consumer_key = TP.getConsumerKey();
string oauth_consumer_secret = TP.getConsumerSecret();
string oauth_token = TP.getToken();
string oauth_token_secret = TP.getTokenSecret();
///////////////////////////////////////////////// FACEBOOK //////////////////////////////////////////////////
string accessToken = "<KEY>";
var objFacebookClient = new FacebookClient(accessToken);
var parameters = new Dictionary<string, object>();
parameters["message"] = message;
/////////////////////////////////////////////////////////////////////////////////////////////////////////////////
Auth.SetUserCredentials(oauth_consumer_key, oauth_consumer_secret, oauth_token, oauth_token_secret);
try
{
objFacebookClient.Post("feed", parameters);
Tweet.PublishTweet(message);
}
catch (Exception ex)
{
LabelResult.ForeColor = System.Drawing.Color.Red;
LabelResult.Visible = true;
LabelResult.Text = ex.Message;
}
}
protected void ButtonTrigger_Click(object sender, EventArgs e)
{
TwitterPerso TP = new TwitterPerso();
string oauth_consumer_key = TP.getConsumerKey();
string oauth_consumer_secret = TP.getConsumerSecret();
string oauth_token = TP.getToken();
string oauth_token_secret = TP.getTokenSecret();
Auth.SetUserCredentials(oauth_consumer_key, oauth_consumer_secret, oauth_token, oauth_token_secret);
var stream = Tweetinvi.Stream.CreateUserStream();
stream.FollowedUser += (senderr, args) =>
{
API sendinBlue = new mailinblue.API("ERWXqjT4mS3aA05V", 5000); //Optional parameter: Timeout in MS
Dictionary<string, Object> data = new Dictionary<string, Object>();
Dictionary<string, string> to = new Dictionary<string, string>();
to.Add("<EMAIL>", "to whom!");
List<string> from_name = new List<string>();
from_name.Add("<EMAIL>");
from_name.Add("AREA.NET");
data.Add("to", to);
data.Add("from", from_name);
data.Add("subject", "AREA send in blue");
data.Add("html", "Pop , you followed " + args.User + " on Twitter :)");
Object sendEmail = sendinBlue.send_email(data);
Tweet.PublishTweet("I followed " + args.User + " on Twitter");
};
stream.StartStream();
}
protected void ButtonUnfollowed_Click(object sender, EventArgs e)
{
TwitterPerso TP = new TwitterPerso();
string oauth_consumer_key = TP.getConsumerKey();
string oauth_consumer_secret = TP.getConsumerSecret();
string oauth_token = TP.getToken();
string oauth_token_secret = TP.getTokenSecret();
Auth.SetUserCredentials(oauth_consumer_key, oauth_consumer_secret, oauth_token, oauth_token_secret);
var stream = Tweetinvi.Stream.CreateUserStream();
stream.UnFollowedUser += (senderr, args) =>
{
string message = "I unfollowed " + args.User + " on Twitter :)";
string accessToken = "<KEY>";
var objFacebookClient = new FacebookClient(accessToken);
var parameters = new Dictionary<string, object>();
parameters["message"] = message;
try
{
Tweet.PublishTweet(message);
objFacebookClient.Post("feed", parameters);
}
catch (FacebookApiException ex)
{
Console.WriteLine(ex.Message);
}
};
stream.StartStream();
}
protected void ButtonDrop_Click(object sender, EventArgs e)
{
TwitterPerso TP = new TwitterPerso();
string oauth_consumer_key = TP.getConsumerKey();
string oauth_consumer_secret = TP.getConsumerSecret();
string oauth_token = TP.getToken();
string oauth_token_secret = TP.getTokenSecret();
Auth.SetUserCredentials(oauth_consumer_key, oauth_consumer_secret, oauth_token, oauth_token_secret);
int i = 0;
var stream = Tweetinvi.Stream.CreateUserStream();
stream.TweetCreatedByMe += (senderr, args) =>
{
DropboxClient DBClient = new DropboxClient("<KEY>");
try
{
string dest = String.Format("D:/Epitech/DOT_AREA_2017/picture({0}).jpg", i++);
var media = args.Tweet.Media[0].MediaURLHttps;
System.Diagnostics.Debug.WriteLine(media);
using (WebClient client = new WebClient())
{
client.DownloadFile(media, dest);
}
using (var streams = new MemoryStream(File.ReadAllBytes(dest)))
{
var response = DBClient.Files.UploadAsync("/area/" + dest, WriteMode.Overwrite.Instance, body: streams);
var result = response.Result;
}
}
catch (Exception ex)
{
LabelResult.Visible = true;
LabelResult.Text = ex.Message;
}
};
stream.StartStream();
}
protected void ButtonTriggerSMS_Click(object sender, EventArgs e)
{
TwitterPerso TP = new TwitterPerso();
string oauth_consumer_key = TP.getConsumerKey();
string oauth_consumer_secret = TP.getConsumerSecret();
string oauth_token = TP.getToken();
string oauth_token_secret = TP.getTokenSecret();
string username = "<EMAIL>";
string password = "<PASSWORD>";
string msgsender = "33683821203";
string destinationaddr = string.Concat("33", TextDestination.Text.Remove(0, 1).ToString());
ViaNettSMS s = new ViaNettSMS(username, password);
ViaNettSMS.Result result;
/////////////////////////////////////////////////////////////////////////////////////////////////////////////////
var stream = Tweetinvi.Stream.CreateUserStream();
stream.BlockedUser += (senderr, args) =>
{
try
{
//Tweet.PublishTweet(message);
result = s.sendSMS(msgsender, destinationaddr, "Hey ! Why you blocked " + args.User + " on Twitter :(");
LabelResult.ForeColor = System.Drawing.Color.Green;
LabelResult.Visible = true;
LabelResult.Text = "Message posted on Twitter and sended to " + TextDestination.Text + " !";
}
catch (Exception ex)
{
LabelResult.ForeColor = System.Drawing.Color.Red;
LabelResult.Visible = true;
LabelResult.Text = ex.Message;
}
};
stream.StartStream();
}
}
}<file_sep>/DOT_cardGames_2017/cardGame/cardGameTests/PlayerTests.cs
using System;
using Microsoft.VisualStudio.TestTools.UnitTesting;
using DotNetty.Transport.Channels;
using System.Collections.Generic;
using cardGame;
namespace cardGameTests
{
[TestClass]
public class PlayerTests
{
IChannelHandlerContext ctx;
[TestMethod]
public void TestGetName()
{
Player player = new Player(ctx, "player_test");
Assert.AreEqual("player_test", player.GetName());
Assert.AreNotEqual("blabla", player.GetName());
Assert.AreNotEqual("zfjzogj", player.GetName());
}
[TestMethod]
public void TestIsBusted()
{
Player player = new Player(ctx, "player_test");
Assert.AreEqual(false, player.IsBusted());
Assert.AreNotEqual(true, player.IsBusted());
}
}
}
<file_sep>/DotNet_epicture_2017/epicture/MainPage.xaml.cs
using Newtonsoft.Json;
using System;
using System.Collections.Generic;
using System.Net.Http;
using System.Threading;
using System.Threading.Tasks;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using System.Diagnostics;
namespace epicture
{
public sealed partial class MainPage : Page
{
Imgur_client client_info;
AutoResetEvent navCompleted;
public MainPage()
{
this.InitializeComponent();
navCompleted = new AutoResetEvent(false);
}
private async void ImgurLogin_OnClick(object sender, RoutedEventArgs e)
{
Uri authUri = new Uri("https://api.imgur.com/oauth2/authorize?&client_id=4c87c24c218e973&response_type=code");
LoginContentFlickr.Visibility = Visibility.Collapsed;
LoginContent.Visibility = Visibility.Collapsed;
ImgurLogin.Visibility = Visibility.Visible;
ImgurLogin.Navigate(authUri);
await Task.Run(() => { navCompleted.WaitOne(); });
navCompleted.Reset();
ImgurLogin.Visibility = Visibility.Collapsed;
ImgurLogin.Visibility = Visibility.Visible;
}
private async void CompletedNav(WebView sender, WebViewNavigationCompletedEventArgs args)
{
String[] res = args.Uri.ToString().Substring(args.Uri.ToString().IndexOf('#') + 1).Split('=');
using (HttpClient client = new HttpClient())
{
var values = new Dictionary<string, string>
{
{ "client_id", "4c87c24c218e973" },
{ "client_secret", "<KEY>" },
{ "grant_type", "authorization_code" },
{ "code", res[1] }
};
FormUrlEncodedContent content = new FormUrlEncodedContent(values);
var response = await client.PostAsync("https://api.imgur.com/oauth2/token", content);
string ret = await response.Content.ReadAsStringAsync();
Debug.WriteLine(ret);
client_info = JsonConvert.DeserializeObject<Imgur_client>(ret);
if (client_info.account_id != 0)
{
this.Frame.Navigate(typeof(HomeImgur), client_info);
}
else
{
Imgur_Request request = JsonConvert.DeserializeObject<Imgur_Request>(ret);
Response.Text = "Error : " + request.data.error;
}
}
}
private void FailedNav(object sender, WebViewNavigationFailedEventArgs e)
{
Response.Visibility = Visibility.Visible;
Response.Text = "Failed to navigate Imgur.";
}
private void FlickrLogin_OnClick(object sender, RoutedEventArgs e)
{
this.Frame.Navigate(typeof(HomeFlickr));
}
private void FailedNavFlickr(object sender, WebViewNavigationFailedEventArgs e)
{
Response.Visibility = Visibility.Visible;
Response.Text = "Failed to navigate Flickr.";
}
private void CompletedNavFlickr(WebView sender, WebViewNavigationCompletedEventArgs args)
{
}
}
}
<file_sep>/DOT_cardGames_2017/cardGame/cardGame/GameState.cs
namespace cardGame
{
enum GameState
{
LOBBY,
GAME,
}
}<file_sep>/DOT_cardGames_2017/cardGame/cardGameTests/CardTests.cs
using System;
using Microsoft.VisualStudio.TestTools.UnitTesting;
using cardGame;
namespace cardGameTests
{
[TestClass]
public class CardTests
{
Card cardH1 = new Card(1, cardGame.Suit.HEART);
Card cardD1 = new Card(1, cardGame.Suit.DIAMOND);
Card cardS1 = new Card(1, cardGame.Suit.SPADE);
Card cardC1 = new Card(1, cardGame.Suit.CLUB);
Card cardH10 = new Card(10, cardGame.Suit.HEART);
Card cardD10 = new Card(10, cardGame.Suit.DIAMOND);
Card cardS10 = new Card(10, cardGame.Suit.SPADE);
Card cardC10 = new Card(10, cardGame.Suit.CLUB);
[TestMethod]
public void TestGetnumber()
{
Assert.AreEqual(1, cardH1.Getnumber());
Assert.AreEqual(1, cardD1.Getnumber());
Assert.AreEqual(1, cardS1.Getnumber());
Assert.AreEqual(1, cardC1.Getnumber());
Assert.AreEqual(10, cardH10.Getnumber());
Assert.AreEqual(10, cardD10.Getnumber());
Assert.AreEqual(10, cardS10.Getnumber());
Assert.AreEqual(10, cardC10.Getnumber());
Assert.AreNotEqual(12, cardH1.Getnumber());
Assert.AreNotEqual(13, cardD1.Getnumber());
Assert.AreNotEqual(14, cardS1.Getnumber());
Assert.AreNotEqual(15, cardC1.Getnumber());
Assert.AreNotEqual(1, cardH10.Getnumber());
Assert.AreNotEqual(2, cardD10.Getnumber());
Assert.AreNotEqual(3, cardS10.Getnumber());
Assert.AreNotEqual(4, cardC10.Getnumber());
}
[TestMethod]
public void TestGetSuit()
{
Assert.AreEqual(Suit.HEART, cardH1.GetSuit());
Assert.AreEqual(Suit.DIAMOND, cardD1.GetSuit());
Assert.AreEqual(Suit.SPADE, cardS1.GetSuit());
Assert.AreEqual(Suit.CLUB, cardC1.GetSuit());
Assert.AreEqual(Suit.HEART, cardH1.GetSuit());
Assert.AreEqual(Suit.DIAMOND, cardD1.GetSuit());
Assert.AreEqual(Suit.SPADE, cardS1.GetSuit());
Assert.AreEqual(Suit.CLUB, cardC1.GetSuit());
Assert.AreNotEqual(Suit.HEART, cardC1.GetSuit());
Assert.AreNotEqual(Suit.DIAMOND, cardS1.GetSuit());
Assert.AreNotEqual(Suit.SPADE, cardD1.GetSuit());
Assert.AreNotEqual(Suit.CLUB, cardH1.GetSuit());
Assert.AreNotEqual(Suit.HEART, cardC1.GetSuit());
Assert.AreNotEqual(Suit.DIAMOND, cardS1.GetSuit());
Assert.AreNotEqual(Suit.SPADE, cardD1.GetSuit());
Assert.AreNotEqual(Suit.CLUB, cardH1.GetSuit());
}
[TestMethod]
public void TestGetCardString()
{
Assert.AreEqual("As of heart", cardH1.GetCardString());
Assert.AreEqual("As of spade", cardS1.GetCardString());
Assert.AreEqual("As of diamond", cardD1.GetCardString());
Assert.AreEqual("As of club", cardC1.GetCardString());
Assert.AreEqual("Ten of heart", cardH10.GetCardString());
Assert.AreEqual("Ten of spade", cardS10.GetCardString());
Assert.AreEqual("Ten of diamond", cardD10.GetCardString());
Assert.AreEqual("Ten of club", cardC10.GetCardString());
Assert.AreNotEqual("As of hddddddeart", cardH1.GetCardString());
Assert.AreNotEqual("As of spadffffffffe", cardS1.GetCardString());
Assert.AreNotEqual("As of diamonddddd", cardD1.GetCardString());
Assert.AreNotEqual("As of clubfffffff", cardC1.GetCardString());
Assert.AreNotEqual("As of hzefzef", cardH10.GetCardString());
Assert.AreNotEqual("As of spadgsdgsdge", cardS10.GetCardString());
Assert.AreNotEqual("As of diamqrgerg", cardD10.GetCardString());
Assert.AreNotEqual("As of clsfsdgsdgb", cardC10.GetCardString());
}
}
}
<file_sep>/DotNet_epicture_2017/epicture/epictureTest/ImgurTest.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Microsoft.VisualStudio.TestTools.UnitTesting;
using epicture;
namespace epictureTest
{
[TestClass]
public class ImgurTest
{
private Imgur_client imgur { get; set; }
[TestInitialize]
public void Initializer()
{
this.imgur = new Imgur_client();
}
[TestMethod]
public void client_info()
{
string id = "4c87c24c218e973";
string sct = "93e8a179129b8393c0256c60a57a0abd40da4b6b";
Assert.AreEqual(this.imgur.app_id, id);
Assert.AreEqual(this.imgur.secret, sct);
}
[TestMethod]
public void username()
{
string name = null;
Assert.AreEqual(this.imgur.account_username, name);
}
[TestMethod]
public void testHttpGet()
{
string x = "false";
Task.Run(async () =>
{
string str = await this.imgur.HttpGet("blabla");
Assert.AreNotEqual(str, x);
});
}
[TestMethod]
public void testHttpDelete()
{
string x = "false";
Task.Run(async () =>
{
string str = await this.imgur.HttpGet("sfsdmlfksdmlfksdfksml");
Assert.AreNotEqual(str, x);
});
}
}
}
<file_sep>/DOT_AREA_2017/DOT_AREA_2017/DOT_AREA_2017/Home/Login.aspx.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Configuration;
using System.Data.SqlClient;
namespace DOT_AREA_2017
{
public partial class Login : Page
{
SqlConnection con = new SqlConnection(ConfigurationManager.ConnectionStrings["ConnectionString"].ConnectionString);
protected void Page_Load(object sender, EventArgs e)
{
con.Open();
}
protected void ButtonLogin_Click(object sender, EventArgs e)
{
try
{
if ((TextLogin.Text != "" && TextLogin != null)
&& (TextPass.Text != "" && TextPass.Text != null))
{
string sql = "select * from [User] where Login=@login AND Password=@<PASSWORD>";
SqlCommand cmd = new SqlCommand(sql, con);
cmd.Parameters.AddWithValue("@login", TextLogin.Text);
cmd.Parameters.AddWithValue("@password", TextPass.Text);
SqlDataReader reader = cmd.ExecuteReader();
if (reader.Read())
{
var url = String.Format("~/Home/Logged.aspx?login={0}&email={1}&username={2}", reader["Login"], reader["Email"], reader["UserName"]);
Response.Redirect(url);
}
else
{
Label1.ForeColor = System.Drawing.Color.Red;
Label1.Visible = true;
Label1.Text = "Invalid login or password ...";
}
reader.Close();
con.Close();
}
else
{
Label1.ForeColor = System.Drawing.Color.Red;
Label1.Visible = true;
Label1.Text = "Invalid login or password ...";
}
}
catch (SqlException ex)
{
Label1.ForeColor = System.Drawing.Color.Red;
Label1.Visible = true;
Label1.Text = ex.Message;
}
}
}
}<file_sep>/DotNet_todolist_2017/todolist/EditTask.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Runtime.InteropServices.WindowsRuntime;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Popups;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
// Pour plus d'informations sur le modèle d'élément Page vierge, consultez la page https://go.microsoft.com/fwlink/?LinkId=234238
namespace todolist
{
/// <summary>
/// Une page vide peut être utilisée seule ou constituer une page de destination au sein d'un frame.
/// </summary>
public sealed partial class EditTask : Page
{
public EditTask()
{
this.InitializeComponent();
App db = App.Current as App;
Title.Text = db.CurrentObj.Title;
ContentContent.Text = db.CurrentObj.Content;
Date.Date = db.CurrentObj.DateT.Date;
}
private async void DeleteTodo_OnClick(object sender, RoutedEventArgs e)
{
string title = "Deleting task";
string content = "Do you want to delete this task ?";
UICommand yesCommand = new UICommand("Yes");
UICommand noCommand = new UICommand("No");
var dialog = new MessageDialog(content, title);
dialog.Options = MessageDialogOptions.None;
dialog.Commands.Add(yesCommand);
dialog.Commands.Add(noCommand);
dialog.DefaultCommandIndex = 0;
dialog.CancelCommandIndex = 0;
IUICommand command = await dialog.ShowAsync();
if (command != noCommand)
{
var db = App.Current as App;
db.connection.Query<TodoDB>("DELETE FROM TodoDB WHERE Id =" + db.CurrentObj.Id);
Frame.Navigate(typeof(MainPage));
}
}
private void BackToMain_OnClick(object sender, RoutedEventArgs e)
{
Frame.Navigate(typeof(MainPage));
}
private void Valid_Click(object sender, RoutedEventArgs e)
{
if (Title.Text.Length == 0)
{
Error.Text = "You must set a title for the task";
Error.Visibility = Visibility.Visible;
return;
}
else if (ContentContent.Text.Length == 0)
{
Error.Text = "You must set a content for the task";
Error.Visibility = Visibility.Visible;
return;
}
else if (!Date.Date.HasValue)
{
Error.Text = "You must choose a date for the task";
Error.Visibility = Visibility.Visible;
return;
}
else
{
App db = App.Current as App;
db.CurrentObj.Title = Title.Text;
db.CurrentObj.DateT = Date.Date.Value;
db.CurrentObj.Content = ContentContent.Text;
db.connection.Update(db.CurrentObj);
Frame.Navigate(typeof(MainPage));
}
}
private async void Delete_Click(object sender, RoutedEventArgs e)
{
string title = "Do you want delete this task ?";
UICommand delete = new UICommand("Delete");
UICommand cancel = new UICommand("Cancel");
MessageDialog option = new MessageDialog(title);
option.Commands.Add(delete);
option.Commands.Add(cancel);
IUICommand command = await option.ShowAsync();
if (command != cancel)
{
App db = App.Current as App;
db.connection.Query<TodoDB>("DELETE FROM TodoDB WHERE Id =" + db.CurrentObj.Id);
}
Frame.Navigate(typeof(MainPage));
}
private void Back_OnClick(object sender, RoutedEventArgs e)
{
Frame.Navigate(typeof(MainPage));
}
}
}
<file_sep>/DOT_cardGames_2017/cardGame/cardGame/Program.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
using System.Security.Cryptography.X509Certificates;
using DotNetty.Codecs;
using DotNetty.Handlers.Logging;
using DotNetty.Handlers.Tls;
using DotNetty.Transport.Bootstrapping;
using DotNetty.Transport.Channels;
using DotNetty.Transport.Channels.Sockets;
using Newtonsoft.Json;
namespace cardGame
{
public class ProtocolJson
{
public string message { get; set; }
public DateTime messageDate { get; set; }
}
class Program
{
static async Task RunServerAsync()
{
var bossGroup = new MultithreadEventLoopGroup(1);
var workerGroup = new MultithreadEventLoopGroup();
var STRING_ENCODER = new StringEncoder();
var STRING_DECODER = new StringDecoder();
var SERVER_HANDLER = new ChatServerHandler();
try
{
var bootstrap = new ServerBootstrap();
bootstrap
.Group(bossGroup, workerGroup)
.Channel<TcpServerSocketChannel>()
.Option(ChannelOption.SoBacklog, 100)
.Handler(new LoggingHandler(LogLevel.INFO))
.ChildHandler(new ActionChannelInitializer<ISocketChannel>(channel =>
{
IChannelPipeline pipeline = channel.Pipeline;
pipeline.AddLast(new DelimiterBasedFrameDecoder(8192, Delimiters.LineDelimiter()));
pipeline.AddLast(STRING_ENCODER, STRING_DECODER, SERVER_HANDLER);
}));
IChannel bootstrapChannel = await bootstrap.BindAsync(8080);
Console.ReadLine();
await bootstrapChannel.CloseAsync();
}
finally
{
Task.WaitAll(bossGroup.ShutdownGracefullyAsync(), workerGroup.ShutdownGracefullyAsync());
}
}
static void Main() => RunServerAsync().Wait();
}
}
<file_sep>/DotNet_epicture_2017/epicture/epictureTest/FlickrTest.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Microsoft.VisualStudio.TestTools.UnitTesting;
using epicture;
namespace epictureTest
{
[TestClass]
public class FlickrTest
{
[TestMethod]
public void Auth()
{
}
}
}
<file_sep>/DOT_cardGames_2017/cardGame/cardGame/Blackjack.cs
using DotNetty.Transport.Channels;
using System;
using System.Collections.Generic;
namespace cardGame
{
public class Blackjack
{
private List<Player> _players;
private int _numberOfPlayer;
private GameState _state;
private Player _playerTurn;
private Dealer _dealer;
private int _numberOfDeck;
public Blackjack()
{
_players = new List<Player>();
_numberOfPlayer = 0;
_state = GameState.LOBBY;
_playerTurn = null;
_dealer = null;
_numberOfDeck = 1;
}
public Card Card
{
get => default(Card);
set
{
}
}
internal GameState GameState
{
get => default(GameState);
set
{
}
}
public Dealer Dealer
{
get => default(Dealer);
set
{
}
}
public Blackjack Blackjack1
{
get => default(Blackjack);
set
{
}
}
public Player Player
{
get => default(Player);
set
{
}
}
private void CommandShowLobby(Player player)
{
foreach (var p in _players)
player.Write(p.GetName());
}
//handle command that can be use at anytime
private bool GeneralCommand(Player player, string cmd)
{
if (string.Equals("showlobby", cmd, StringComparison.OrdinalIgnoreCase))
CommandShowLobby(player);
else
return false;
return true;
}
//Start a new turn of blackjack
private void HandOutTwoCardToAll()
{
_dealer.ClearHand();
foreach (var p in _players)
p.ClearHand();
_dealer.DealCard(null);
foreach (var p in _players)
_dealer.DealCard(p);
_dealer.DealCard(null);
foreach (var p in _players)
_dealer.DealCard(p);
}
//print cards to all player
private void DisplayTurnStateToAllPlayer()
{
foreach (var p in _players)
_dealer.DisplayHideHandToPlayer(p);
foreach (var p in _players)
p.DisplayHand();
}
//print "it is player's turn"
public void DisplayPlayerTurnToAll()
{
foreach (var p in _players)
{
if (p != _playerTurn)
p.Write("It is " + _playerTurn.GetName() + "'s turn");
}
}
//Start a new turn of black from "start" command
private void InitiateNewGame(Player player)
{
_dealer = new Dealer(_numberOfDeck);
_playerTurn = player;
HandOutTwoCardToAll();
DisplayTurnStateToAllPlayer();
DisplayPlayerTurnToAll();
_playerTurn.AskPlayerAction();
}
private void CommandStart(Player player)
{
_state = GameState.GAME;
_playerTurn = player;
InitiateNewGame(player);
}
private void CommandDeck(Player player, string cmd)
{
int number = 1;
if (Int32.TryParse(cmd, out number) == false)
player.InvalidCommand();
else if (number < 1 || number > 4)
player.Write("You can play with 1 to 4 deck only");
else
{
_numberOfDeck = number;
player.Write("Number of deck set to " + _numberOfDeck);
}
}
//handle command from lobby
private void LobbyCommand(Player player, string cmd)
{
if (string.Equals("start", cmd, StringComparison.OrdinalIgnoreCase))
CommandStart(player);
else if (cmd.ToLower().StartsWith("deck ") == true)
CommandDeck(player, cmd.Substring(5));
else
player.InvalidCommand();
}
private int GetPlayerByIndex(Player player)
{
int i = 0;
for (i = 0; i < _players.Count; i++)
if (_players[i] == player)
break;
return i;
}
private void SetNextPlayerturn()
{
int index = GetPlayerByIndex(_playerTurn);
int max = _players.Count;
Player next;
if (index >= max - 1)
next = _players[0];
else
next = _players[index + 1];
_playerTurn = next;
if (_playerTurn.IsHandEmpty() == true && _players.Count > 1)
{
SetNextPlayerturn();
}
}
private bool IsEndTurn()
{
foreach (var p in _players)
if (p.GetHasPlay() == false && p.IsHandEmpty() == false)
return false;
return true;
}
private void StartNewTurn()
{
foreach (var p in _players)
{
p.SetHasPlay(false);
p.Write("Starting new turn !");
}
if (_dealer.GetShoeCount() < 15)
{
_dealer.ClearShoe();
_dealer.GenerateShoe();
}
HandOutTwoCardToAll();
DisplayTurnStateToAllPlayer();
DisplayPlayerTurnToAll();
_playerTurn.AskPlayerAction();
}
private void CalculateWinners()
{
if (_dealer.IsBusted())
{
foreach (var p in _players)
{
if (p.IsHandEmpty() == false)
{
if (p.IsBusted())
p.Write("You lost this hand");
else
p.Write("You won this hand !");
}
}
}
else
{
foreach (var p in _players)
{
if (p.IsHandEmpty() == false)
{
if (p.IsBusted() || p.GetHandValue() < _dealer.GetHandValue())
p.Write("You lost this hand");
else if (p.GetHandValue() == _dealer.GetHandValue())
p.Write("Draw");
else
p.Write("You won this hand !");
}
}
}
}
private void DealerTurn()
{
foreach (var p in _players)
{
if (p.IsHandEmpty() == false)
{
p.Write("Dealer's turn");
_dealer.DisplayHandToPlayer(p, true);
}
}
while (_dealer.GetHandValue() < 17)
{
_dealer.DealCard(null);
foreach (var p in _players)
{
if (p.IsHandEmpty() == false)
_dealer.DisplayHandToPlayer(p, true);
}
}
}
private void CommandHit(Player player)
{
_dealer.DealCard(player);
player.DisplayHand();
if (player.IsBusted() == true)
{
player.Write("Busted !");
player.SetHasPlay(true);
SetNextPlayerturn();
if (IsEndTurn() == true)
{
DealerTurn();
CalculateWinners();
StartNewTurn();
}
else
{
DisplayPlayerTurnToAll();
_playerTurn.AskPlayerAction();
}
}
else
_playerTurn.AskPlayerAction();
}
private void CommandStand(Player player)
{
player.SetHasPlay(true);
SetNextPlayerturn();
if (IsEndTurn() == true)
{
DealerTurn();
CalculateWinners();
StartNewTurn();
}
else
{
DisplayPlayerTurnToAll();
_playerTurn.AskPlayerAction();
}
}
private void CommandDouble(Player player)
{
_dealer.DealCard(player);
player.DisplayHand();
player.SetHasPlay(true);
SetNextPlayerturn();
if (player.IsBusted() == true)
player.Write("Busted !");
if (IsEndTurn() == true)
{
DealerTurn();
CalculateWinners();
StartNewTurn();
}
else
{
DisplayPlayerTurnToAll();
_playerTurn.AskPlayerAction();
}
}
//handle command related to ingame blackjack
private void GameCommand(Player player, string cmd)
{
if (player != _playerTurn)
player.InvalidCommand();
else if (string.Equals("hit", cmd, StringComparison.OrdinalIgnoreCase))
CommandHit(player);
else if (string.Equals("stand", cmd, StringComparison.OrdinalIgnoreCase))
CommandStand(player);
else if (string.Equals("double", cmd, StringComparison.OrdinalIgnoreCase))
CommandDouble(player);
else
player.InvalidCommand();
}
//handle command related to blackjack
private void BlackjackCommand(Player player, string cmd)
{
if (_state == GameState.LOBBY)
LobbyCommand(player, cmd);
else if (_state == GameState.GAME)
GameCommand(player, cmd);
}
// main function
public void BlackjackHandler(IChannelHandlerContext contex, string cmd)
{
Player player = GetPlayerByContex(contex);
if (GeneralCommand(player, cmd) == false)
BlackjackCommand(player, cmd);
}
public void AddPlayer(IChannelHandlerContext contex)
{
string name = "Player " + _numberOfPlayer++;
Player player = new Player(contex, name);
foreach (var p in _players)
{
if (p != player)
p.Write(player.GetName() + " has join");
}
_players.Add(player);
if (_state == GameState.GAME)
{
player.Write("Wait for next turn");
_playerTurn.AskPlayerAction();
}
}
public Player GetPlayerByContex(IChannelHandlerContext contex)
{
Player player = null;
foreach (var p in _players)
{
if (p.GetContex() == contex)
{
player = p;
break;
}
}
return player;
}
public void RemovePlayerByContex(IChannelHandlerContext contex)
{
Player player = GetPlayerByContex(contex);
foreach (var p in _players)
{
if (player != p)
p.Write(player.GetName() + " has left");
}
_players.Remove(player);
if (_players.Count == 0)
_state = GameState.LOBBY;
if (_state == GameState.GAME)
{
SetNextPlayerturn();
if (IsEndTurn() == true)
{
DealerTurn();
CalculateWinners();
StartNewTurn();
}
else
{
DisplayPlayerTurnToAll();
_playerTurn.AskPlayerAction();
}
}
}
}
}<file_sep>/DOT_cardGames_2017/cardGame/client/Program.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
using System.Net;
using System.Net.Security;
using System.Security.Cryptography.X509Certificates;
using DotNetty.Codecs;
using DotNetty.Handlers.Logging;
using DotNetty.Handlers.Tls;
using DotNetty.Transport.Bootstrapping;
using DotNetty.Transport.Channels;
using DotNetty.Transport.Channels.Sockets;
using Newtonsoft.Json;
namespace client
{
public class ProtocolJson
{
public string message { get; set; }
public DateTime messageDate { get; set; }
}
class Program
{
static async Task RunClientAsync()
{
var HOST = IPAddress.Parse("127.0.0.1");
var group = new MultithreadEventLoopGroup();
try
{
var bootstrap = new Bootstrap();
bootstrap
.Group(group)
.Channel<TcpSocketChannel>()
.Option(ChannelOption.TcpNodelay, true)
.Handler(new ActionChannelInitializer<ISocketChannel>(channel =>
{
IChannelPipeline pipeline = channel.Pipeline;
pipeline.AddLast(new DelimiterBasedFrameDecoder(8192, Delimiters.LineDelimiter()));
pipeline.AddLast(new StringEncoder(), new StringDecoder(), new ChatClientHandler());
}));
IChannel bootstrapChannel = await bootstrap.ConnectAsync(new IPEndPoint(HOST, 8080));
ProtocolJson pj = new ProtocolJson();
for (;;)
{
string line = Console.ReadLine();
pj.message = line;
pj.messageDate = DateTime.Now;
string Json = JsonConvert.SerializeObject(pj, Formatting.None);
if (string.IsNullOrEmpty(line))
{
continue;
}
try
{
await bootstrapChannel.WriteAndFlushAsync(Json + "\r\n");
//await bootstrapChannel.WriteAndFlushAsync(line + "\r\n");
}
catch
{
}
if (string.Equals(line, "bye", StringComparison.OrdinalIgnoreCase))
{
await bootstrapChannel.CloseAsync();
break;
}
}
await bootstrapChannel.CloseAsync();
}
finally
{
group.ShutdownGracefullyAsync().Wait(1000);
}
}
static void Main() => RunClientAsync().Wait();
}
}
<file_sep>/DOT_AREA_2017/DOT_AREA_2017/DOT_AREA_2017/Home/Logged.aspx.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
namespace DOT_AREA_2017
{
public partial class Logged : Page
{
protected void Page_Load(object sender, EventArgs e)
{
var login = Request["login"];
var email = Request["email"];
var username = Request["username"];
LabelUsername.Visible = true;
LabelUsername.Text = String.Format("Welcome {0}", login);
}
}
}<file_sep>/DOT_cardGames_2017/cardGame/client/ChatClientHandler.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using DotNetty.Transport.Channels;
using Newtonsoft.Json;
namespace client
{
public class ChatClientHandler : SimpleChannelInboundHandler<string>
{
internal Program Program
{
get => default(Program);
set
{
}
}
protected override void ChannelRead0(IChannelHandlerContext contex, string msg)
{
Console.WriteLine(msg);
}
public override void ExceptionCaught(IChannelHandlerContext contex, Exception e)
{
Console.WriteLine(DateTime.Now.Millisecond);
Console.WriteLine(e.StackTrace);
contex.CloseAsync();
}
}
}
<file_sep>/DOT_AREA_2017/DOT_AREA_2017/DOT_AREA_2017/Home/API/Facebook.aspx.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using mailinblue;
using Vianett.Common.Log4net;
using System.Net;
using System.IO;
using System.Security.Cryptography;
using System.Text;
using Facebook;
namespace DOT_AREA_2017
{
public partial class Facebook : Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
protected void ButtonMail_Click(object sender, EventArgs e)
{
string message = TextFacebook.InnerText;
///////////////////////////////////////////////// SEND IN BLUE //////////////////////////////////////////////////
API sendinBlue = new mailinblue.API("ERWXqjT4mS3aA05V", 5000); //Optional parameter: Timeout in MS
Dictionary<string, Object> data = new Dictionary<string, Object>();
Dictionary<string, string> to = new Dictionary<string, string>();
to.Add(TextDestination.Text, "to whom!");
List<string> from_name = new List<string>();
from_name.Add("<EMAIL>");
from_name.Add("AREA.NET");
//List<string> attachment = new List<string>();
//attachment.Add("https://domain.com/path-to-file/filename1.pdf");
//attachment.Add("https://domain.com/path-to-file/filename2.jpg");
data.Add("to", to);
data.Add("from", from_name);
data.Add("subject", "AREA send in blue");
data.Add("html", String.Concat("You post the following message on Facebook : ", message));
//data.Add("attachment", attachment);
Object sendEmail = sendinBlue.send_email(data);
/////////////////////////////////////////////////////////////////////////////////////////////////////////////////
string accessToken = "<KEY>";
var objFacebookClient = new FacebookClient(accessToken);
var parameters = new Dictionary<string, object>();
parameters["message"] = message;
try
{
objFacebookClient.Post("feed", parameters);
LabelResult.ForeColor = System.Drawing.Color.Green;
LabelResult.Visible = true;
LabelResult.Text = "Message posted on Facebook and sended to " + TextDestination.Text + " !";
}
catch (FacebookApiException fbex)
{
LabelResult.Visible = true;
LabelResult.Text = fbex.Message;
}
}
protected void ButtonPhone_Click(object sender, EventArgs e)
{
string message = TextFacebook.InnerText;
string accessToken = "<KEY>";
var objFacebookClient = new FacebookClient(accessToken);
var parameters = new Dictionary<string, object>();
parameters["message"] = message;
//////////////////////////////////////////// VIANETT /////////////////////////////////////////
string username = "<EMAIL>";
string password = "<PASSWORD>";
string msgsender = "33683821203";
string destinationaddr = string.Concat("33", TextDestination.Text.Remove(0, 1).ToString());
ViaNettSMS s = new ViaNettSMS(username, password);
ViaNettSMS.Result result;
try
{
objFacebookClient.Post("feed", parameters);
result = s.sendSMS(msgsender, destinationaddr, String.Concat("You post on Facebook : ", message));
LabelResult.ForeColor = System.Drawing.Color.Green;
LabelResult.Visible = true;
LabelResult.Text = "Message posted on Facebook and sended to " + TextDestination.Text + " !";
}
catch (FacebookApiException fbex)
{
LabelResult.Visible = true;
LabelResult.Text = fbex.Message;
}
}
protected void ButtonFb_Click(object sender, EventArgs e)
{
string message = TextFacebook.InnerText;
//The facebook json url to update the status
string twitterURL = "https://api.twitter.com/1.1/statuses/update.json";
//set the access tokens (REQUIRED)
string oauth_consumer_key = "OJiBjYwsTTPUHpgIpGbdDfoZZ";
string oauth_consumer_secret = "<KEY>";
string oauth_token = "<KEY>";
string oauth_token_secret = "<KEY>";
// set the oauth version and signature method
string oauth_version = "1.0";
string oauth_signature_method = "HMAC-SHA1";
// create unique request details
string oauth_nonce = Convert.ToBase64String(new ASCIIEncoding().GetBytes(DateTime.Now.Ticks.ToString()));
System.TimeSpan timeSpan = (DateTime.UtcNow - new DateTime(1970, 1, 1, 0, 0, 0, 0, DateTimeKind.Utc));
string oauth_timestamp = Convert.ToInt64(timeSpan.TotalSeconds).ToString();
// create oauth signature
string baseFormat = "oauth_consumer_key={0}&oauth_nonce={1}&oauth_signature_method={2}" + "&oauth_timestamp={3}&oauth_token={4}&oauth_version={5}&status={6}";
string baseString = string.Format(
baseFormat,
oauth_consumer_key,
oauth_nonce,
oauth_signature_method,
oauth_timestamp, oauth_token,
oauth_version,
Uri.EscapeDataString(message)
);
string oauth_signature = null;
using (HMACSHA1 hasher = new HMACSHA1(ASCIIEncoding.ASCII.GetBytes(Uri.EscapeDataString(oauth_consumer_secret) + "&" + Uri.EscapeDataString(oauth_token_secret))))
{
oauth_signature = Convert.ToBase64String(hasher.ComputeHash(ASCIIEncoding.ASCII.GetBytes("POST&" + Uri.EscapeDataString(twitterURL) + "&" + Uri.EscapeDataString(baseString))));
}
// create the request header
string authorizationFormat = "OAuth oauth_consumer_key=\"{0}\", oauth_nonce=\"{1}\", " + "oauth_signature=\"{2}\", oauth_signature_method=\"{3}\", " + "oauth_timestamp=\"{4}\", oauth_token=\"{5}\", " + "oauth_version=\"{6}\"";
string authorizationHeader = string.Format(
authorizationFormat,
Uri.EscapeDataString(oauth_consumer_key),
Uri.EscapeDataString(oauth_nonce),
Uri.EscapeDataString(oauth_signature),
Uri.EscapeDataString(oauth_signature_method),
Uri.EscapeDataString(oauth_timestamp),
Uri.EscapeDataString(oauth_token),
Uri.EscapeDataString(oauth_version)
);
HttpWebRequest objHttpWebRequest = (HttpWebRequest)WebRequest.Create(twitterURL);
objHttpWebRequest.Headers.Add("Authorization", authorizationHeader);
objHttpWebRequest.Method = "POST";
objHttpWebRequest.ContentType = "application/x-www-form-urlencoded";
using (Stream objStream = objHttpWebRequest.GetRequestStream())
{
byte[] content = ASCIIEncoding.ASCII.GetBytes("status=" + Uri.EscapeDataString(message));
objStream.Write(content, 0, content.Length);
}
var responseResult = "";
///////////////////////////////////////////////// FACEBOOK //////////////////////////////////////////////////
string accessToken = "<KEY>";
var objFacebookClient = new FacebookClient(accessToken);
var parameters = new Dictionary<string, object>();
parameters["message"] = message;
/////////////////////////////////////////////////////////////////////////////////////////////////////////////////
try
{
objFacebookClient.Post("feed", parameters);
//success posting
WebResponse objWebResponse = objHttpWebRequest.GetResponse();
StreamReader objStreamReader = new StreamReader(objWebResponse.GetResponseStream());
responseResult = objStreamReader.ReadToEnd().ToString();
}
catch (FacebookApiException fbex)
{
LabelResult.Visible = true;
LabelResult.Text = fbex.Message;
}
catch (Exception ex)
{
//throw exception error
responseResult = "Twitter Post Error: " + ex.Message.ToString() + ", authHeader: " + authorizationHeader;
}
}
}
}<file_sep>/DOT_cardGames_2017/cardGame/cardGameTests/BlackjackTests.cs
using Microsoft.VisualStudio.TestTools.UnitTesting;
using cardGame;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace cardGame.Tests
{
[TestClass()]
public class BlackjackTests
{
Blackjack bj = new Blackjack();
[TestMethod()]
public void GetPlayerByIndexTest()
{
}
}
}<file_sep>/DOT_cardGames_2017/cardGame/cardGame/Card.cs
using System.Collections.Generic;
namespace cardGame
{
public class Card
{
private int _number;
private int _value;
private Suit _suit;
private Dictionary<int, string> _dico;
public Card(int number, Suit suit)
{
_number = number;
_suit = suit;
_value = (number > 10 ? 10 : number);
_dico = new Dictionary<int, string>()
{
{ 1, "As"},
{ 2, "Two"},
{ 3, "Three"},
{ 4, "Four"},
{ 5, "Five"},
{ 6, "Six"},
{ 7, "Seven"},
{ 8, "Eight"},
{ 9, "Nine"},
{ 10, "Ten"},
{ 11, "Jack"},
{ 12, "Queen"},
{ 13, "King"},
};
}
public Suit Suit
{
get => default(Suit);
set
{
}
}
public int Getnumber()
{
return _number;
}
public int GetValue()
{
return _value;
}
public Suit GetSuit()
{
return _suit;
}
public string GetCardString()
{
string str = "";
str += _dico[_number] + " of " + _suit.ToString().ToLower();
return str;
}
}
}<file_sep>/DotNet_epicture_2017/epicture/epictureTest/UnitTest.cs
using System;
using Microsoft.VisualStudio.TestTools.UnitTesting;
using epicture;
namespace epictureTest
{
[TestClass]
public class UnitTest1
{
}
}
<file_sep>/DOT_cardGames_2017/cardGame/cardGame/Dealer.cs
using System;
using System.Collections.Generic;
using System.Security.Cryptography;
namespace cardGame
{
public class Dealer
{
private List<Card> _shoe = new List<Card>();
private Hand _hand = new Hand();
private int _numberOfDeck;
public Dealer(int numberOfDeck)
{
_numberOfDeck = numberOfDeck;
GenerateShoe();
}
public Card Card
{
get => default(Card);
set
{
}
}
public void ClearShoe() => _shoe.Clear();
//Create a new shoe from "_numberOfDeck"
public void GenerateShoe()
{
for (int count = 0; count < _numberOfDeck; count++)
{
for (int i = 1; i <= 13; i++)
_shoe.Add(new Card(i, Suit.HEART));
for (int i = 1; i <= 13; i++)
_shoe.Add(new Card(i, Suit.SPADE));
for (int i = 1; i <= 13; i++)
_shoe.Add(new Card(i, Suit.DIAMOND));
for (int i = 1; i <= 13; i++)
_shoe.Add(new Card(i, Suit.CLUB));
}
Shuffle();
}
public void Shuffle()
{
RNGCryptoServiceProvider provider = new RNGCryptoServiceProvider();
int n = _shoe.Count;
while (n > 1)
{
byte[] box = new byte[1];
do provider.GetBytes(box);
while (!(box[0] < n * (Byte.MaxValue / n)));
int k = (box[0] % n);
n--;
Card card = _shoe[k];
_shoe[k] = _shoe[n];
_shoe[n] = card;
}
}
public void DealCard(Player player, int index = 0)
{
Card card = _shoe[0];
_shoe.RemoveAt(0);
if (player == null)
{
TakeCard(card);
}
else
{
player.TakeCard(card, index);
}
}
public int GetShoeCount() => _shoe.Count;
public void TakeCard(Card card) => _hand.TakeCard(card);
public void ClearHand(int index = 0)
{
_hand.ClearHand(index);
}
public int GetHandValue(int index = 0) => _hand.GetHandValue(index);
public void DisplayHandToPlayer(Player player, bool dealer = false) => player.Write(_hand.GetDisplayHandMsg(0, dealer));
public void DisplayHideHandToPlayer(Player player) => player.Write(_hand.GetDisplayHidHandMsg());
public bool IsBusted() => _hand.IsBusted();
}
}<file_sep>/DOT_AREA_2017/DOT_AREA_2017/DOT_AREA_2017/Home/Others/TwitterPerso.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace DOT_AREA_2017.Home.Others
{
public class TwitterPerso
{
private string oauth_consumer_key = "Cq9CVm4dCDhD3hZASE37ddWP1";
private string oauth_consumer_secret = "<KEY>";
private string oauth_token = "<KEY>";
private string oauth_token_secret = "<KEY>";
public TwitterPerso()
{
}
public string getConsumerKey()
{
return (this.oauth_consumer_key);
}
public string getConsumerSecret()
{
return (this.oauth_consumer_secret);
}
public string getToken()
{
return (this.oauth_token);
}
public string getTokenSecret()
{
return (this.oauth_token_secret);
}
}
}<file_sep>/DotNet_todolist_2017/todolist/AddTask.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Runtime.InteropServices.WindowsRuntime;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
// Pour plus d'informations sur le modèle d'élément Page vierge, consultez la page https://go.microsoft.com/fwlink/?LinkId=234238
namespace todolist
{
/// <summary>
/// Une page vide peut être utilisée seule ou constituer une page de destination au sein d'un frame.
/// </summary>
public sealed partial class AddTask : Page
{
public AddTask()
{
this.InitializeComponent();
}
/*
private void Back_OnClick(object sender, RoutedEventArgs e)
{
Frame.Navigate(typeof(MainPage));
}
*/
private void Valid_Click(object sender, RoutedEventArgs e)
{
App db = App.Current as App;
if (TitleContent.Text.Length == 0)
{
parseCheck.Text = "You must set a title for the task";
parseCheck.Visibility = Visibility.Visible;
return;
}
else if (ContentContent.Text.Length == 0)
{
parseCheck.Text = "You must set a content for the task.";
parseCheck.Visibility = Visibility.Visible;
return;
}
else if (!DueDate.Date.HasValue)
{
parseCheck.Text = "You must choose a date for the task.";
parseCheck.Visibility = Visibility.Visible;
return;
}
else
{
db.connection.Insert(new TodoDB()
{
Title = TitleContent.Text,
DateT = DueDate.Date.Value,
Content = ContentContent.Text,
Status = !true,
});
Frame.Navigate(typeof(MainPage));
}
}
private void Return_OnClick(object sender, RoutedEventArgs e)
{
Frame.Navigate(typeof(MainPage));
}
}
}
<file_sep>/DOT_AREA_2017/DOT_AREA_2017/DOT_AREA_2017/Home/Register.aspx.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data.SqlClient;
using System.Configuration;
namespace DOT_AREA_2017
{
public partial class Register : Page
{
SqlConnection con = new SqlConnection(ConfigurationManager.ConnectionStrings["ConnectionString"].ConnectionString);
protected void Page_Load(object sender, EventArgs e)
{
con.Open();
}
protected void ButtonRegister_Click(object sender, EventArgs e)
{
try
{
if (TextLogin.Text != "" && TextLogin != null)
{
string sql = "insert into [User] (Login,Password,Email,UserName) VALUES(@login,@password,@email,@username)";
SqlCommand cmd = new SqlCommand(sql, con);
cmd.Parameters.AddWithValue("@login", TextLogin.Text);
cmd.Parameters.AddWithValue("@password", <PASSWORD>);
cmd.Parameters.AddWithValue("@email", TextEmail.Text);
cmd.Parameters.AddWithValue("@username", TextUser.Text);
cmd.ExecuteNonQuery();
con.Close();
Label1.Visible = true;
Label1.Text = "Registration successful !";
TextLogin.Text = "";
TextPass.Text = "";
TextEmail.Text = "";
TextUser.Text = "";
}
else
{
Label1.ForeColor = System.Drawing.Color.Red;
Label1.Visible = true;
Label1.Text = "ERROR FORM";
}
}
catch (SqlException ex)
{
Label1.ForeColor = System.Drawing.Color.Red;
Label1.Visible = true;
Label1.Text = ex.Message.ToString();
}
}
protected void SqlDataSource1_Selecting(object sender, SqlDataSourceSelectingEventArgs e)
{
}
}
}<file_sep>/DotNet_todolist_2017/todolist/MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Runtime.InteropServices.WindowsRuntime;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI;
using Windows.UI.Popups;
using Windows.UI.Text;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using SQLite.Net.Attributes;
// Pour plus d'informations sur le modèle d'élément Page vierge, consultez la page https://go.microsoft.com/fwlink/?LinkId=402352&clcid=0x409
namespace todolist
{
public class Task : Grid
{
public int Id { get; set; }
public string Title { get; set; }
public DateTimeOffset DateT { get; set; }
public string Content { get; set; }
public bool Status { get; set; }
}
public class TodoDB
{
[PrimaryKey, AutoIncrement]
public int Id { get; set; }
public string Title { get; set; }
public DateTimeOffset DateT { get; set; }
public string Content { get; set; }
public bool Status { get; set; }
}
/// <summary>
/// Une page vide peut être utilisée seule ou constituer une page de destination au sein d'un frame.
/// </summary>
public sealed partial class MainPage : Page
{
public Task actualTask;
public static DateTimeOffset dateTmp = DateTimeOffset.Now;
public MainPage()
{
this.InitializeComponent();
string path = Path.Combine(Windows.Storage.ApplicationData.Current.LocalFolder.Path, "dbtodo.sqlite");
App db = App.Current as App;
db.connection = new SQLite.Net.SQLiteConnection(new SQLite.Net.Platform.WinRT.SQLitePlatformWinRT(), path);
db.connection.CreateTable<TodoDB>();
}
private void reload(object sender, RoutedEventArgs e)
{
App db = App.Current as App;
SQLite.Net.TableQuery<TodoDB> task = db.connection.Table<TodoDB>();
foreach (TodoDB theTask in task)
{
Grid pos;
TextBlock date;
TextBlock message;
TodoList.Children.Add(pos = new Task
{
Background = new SolidColorBrush(Colors.Black),
Margin = new Thickness(50, 50, 0, 0),
Width = 200,
Height = 200,
Id = theTask.Id,
Status = theTask.Status
});
pos.Children.Add(new TextBlock
{
Text = theTask.Title,
Foreground = new SolidColorBrush(Colors.White),
TextAlignment = TextAlignment.Center,
FontWeight = FontWeights.ExtraBlack,
TextWrapping = TextWrapping.Wrap,
MaxHeight = 50,
Margin = new Thickness(0, 0, 0, 75)
});
pos.Children.Add(date = new TextBlock
{
TextWrapping = TextWrapping.Wrap,
HorizontalAlignment = HorizontalAlignment.Center,
Margin = new Thickness(0, 75, 0, 0),
Text = theTask.DateT.ToString("d"),
Foreground = new SolidColorBrush(Colors.White),
FontWeight = FontWeights.ExtraBlack,
});
pos.Children.Add(message = new TextBlock
{
TextAlignment = TextAlignment.Center,
TextWrapping = TextWrapping.Wrap,
Margin = new Thickness(0, 125, 0, 0),
Visibility = Visibility.Collapsed
});
if (theTask.Status)
{
message.Text = "Finished";
message.FontSize = 30;
message.Foreground = new SolidColorBrush(Colors.LightGreen);
message.Visibility = Visibility.Visible;
}
pos.PointerPressed += new PointerEventHandler(Task_OnClick);
}
}
private void Task_OnClick(object obj, PointerRoutedEventArgs ev)
{
if (this.actualTask != null)
this.actualTask.Background = new SolidColorBrush(Colors.Black);
Edit.Visibility = Visibility.Visible;
Delete.Visibility = Visibility.Visible;
ChangeStatus.Visibility = Visibility.Visible;
this.actualTask = (Task)obj;
this.actualTask.Background = new SolidColorBrush(Colors.DarkGray);
}
/*
private void dueDate_OnDateChanged(CalendarDatePicker datePicker, CalendarDatePickerDateChangedEventArgs dateChanged)
{
if (dateTmp == dateChanged.NewDate)
return;
dateTmp = dateChanged.OldDate.Value;
datePicker.Date = dateChanged.OldDate;
}*/
private void Add_Click(object sender, RoutedEventArgs e)
{
Frame.Navigate(typeof(AddTask));
}
private void Status_Click(object sender, RoutedEventArgs e)
{
App db = App.Current as App;
TodoDB status = db.connection.Query<TodoDB>("SELECT * FROM TodoDB WHERE Id =" + this.actualTask.Id).FirstOrDefault();
//status.Status = !status.Status;
if (status.Status)
{
status.Status = false;
}
else
{
status.Status = true;
}
db.connection.Update(status);
Frame.Navigate(typeof(MainPage));
}
private async void Delete_Click(object sender, RoutedEventArgs e)
{
string title = "Do you want delete this task ?";
UICommand delete = new UICommand("Delete");
UICommand cancel = new UICommand("Cancel");
MessageDialog dialogMessage = new MessageDialog(title);
dialogMessage.Commands.Add(delete);
dialogMessage.Commands.Add(cancel);
IUICommand command = await dialogMessage.ShowAsync();
if (command != cancel)
{
App db = App.Current as App;
db.connection.Query<TodoDB>("DELETE FROM TodoDB WHERE Id =" + this.actualTask.Id);
}
Frame.Navigate(typeof(MainPage));
}
private void Edit_Click(object sender, RoutedEventArgs e)
{
App db = App.Current as App;
db.CurrentObj = db.connection.Query<TodoDB>("SELECT * FROM TodoDB WHERE Id =" + this.actualTask.Id).FirstOrDefault();
Frame.Navigate(typeof(EditTask));
}
}
}
<file_sep>/DOT_cardGames_2017/cardGame/cardGame/ChatServerHandler.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Net;
using DotNetty.Transport.Channels;
using DotNetty.Transport.Channels.Groups;
using Newtonsoft.Json;
namespace cardGame
{
public class ChatServerHandler : SimpleChannelInboundHandler<string>
{
static volatile IChannelGroup group;
private static Blackjack _bj = new Blackjack();
public override void ChannelActive(IChannelHandlerContext contex)
{
IChannelGroup g = group;
if (g == null)
{
lock (this)
{
if (group == null)
{
g = group = new DefaultChannelGroup(contex.Executor);
}
}
}
contex.WriteAndFlushAsync("Welcome to BLACKJACK !\r\n");
g.Add(contex.Channel);
_bj.AddPlayer(contex);
}
class EveryOneBut : IChannelMatcher
{
readonly IChannelId id;
public EveryOneBut(IChannelId id)
{
this.id = id;
}
public bool Matches(IChannel channel) => channel.Id != this.id;
}
protected override void ChannelRead0(IChannelHandlerContext contex, string msg)
{
ProtocolJson pj = JsonConvert.DeserializeObject<ProtocolJson>(msg);
Console.WriteLine("Server receive : " + pj.message + "\n at : " + pj.messageDate + "\n from : " + contex.Channel.RemoteAddress);
//send message to all but this one
//string broadcast = string.Format("[{0}] {1}\n", contex.Channel.RemoteAddress, pj.message);
//string response = string.Format("[you] {0}\n", pj.message);
//group.WriteAndFlushAsync(broadcast, new EveryOneBut(contex.Channel.Id));
//contex.WriteAndFlushAsync(response);
if (string.Equals("bye", pj.message, StringComparison.OrdinalIgnoreCase))
{
_bj.RemovePlayerByContex(contex);
contex.CloseAsync();
}
else
{
_bj.BlackjackHandler(contex, pj.message);
}
}
public override void ChannelReadComplete(IChannelHandlerContext ctx) => ctx.Flush();
public override void ExceptionCaught(IChannelHandlerContext ctx, Exception e)
{
Console.WriteLine("{0}", e.StackTrace);
ctx.CloseAsync();
}
public override bool IsSharable => true;
internal Program Program
{
get => default(Program);
set
{
}
}
}
}
<file_sep>/DOT_cardGames_2017/cardGame/cardGame/Player.cs
using DotNetty.Transport.Channels;
using System.Collections.Generic;
namespace cardGame
{
public class Player
{
private IChannelHandlerContext _contex;
private string _name;
private bool _hasPlay;
private Hand _hand = new Hand();
public Player(IChannelHandlerContext contex, string name)
{
_contex = contex;
_name = name;
_hasPlay = false;
}
public Hand Hand
{
get => default(Hand);
set
{
}
}
public IChannelHandlerContext GetContex() => _contex;
public string GetName() => _name;
public void Write(string msg) => _contex.WriteAndFlushAsync("[Server]: " + msg + "\r\n");
public void InvalidCommand() => _contex.WriteAndFlushAsync("[Server]: invalid command\r\n");
public void TakeCard(Card card, int index = 0) => _hand.TakeCard(card, index);
public void ClearHand() => _hand.ClearHand();
public int GetHandValue(int index = 0) => _hand.GetHandValue(index);
public void DisplayHand(int index = 0) => Write(_hand.GetDisplayHandMsg(index));
public bool IsBusted(int index = 0) => _hand.IsBusted(index);
public List<Card> GetHand(int index = 0) => _hand.GetHand(index);
public bool GetHasPlay() => _hasPlay;
public void SetHasPlay(bool b) => _hasPlay = b;
public void SplitCard(int index = 0) => _hand.SplitCard(index);
//Ask what to do
public void AskPlayerAction(int index = 0)
{
Write(_hand.GetPlayerActionMsg());
}
public bool IsHandEmpty()
{
return _hand.IsEmpty();
}
}
} | be20523e3a8b134db57e105e20f591c5b0f2e580 | [
"C#"
] | 26 | C# | Clemsx/Csharp | 4fd6b69a8fe1c7edfb322698ba9fd2081901f257 | 203279a7e92f4eb6ef20dd44845b6019cf72e757 | |
refs/heads/master | <repo_name>revcozmo/racer<file_sep>/js/car.js
var Car = function(game) {
this.game = game;
this.offsetX = 0;
this.offsetY = 0;
this.mileage = 0;
this.z = this.game.cameraDepth * this.game.pseudo3DCamera.y / (this.game.resolution.y / 2);
this.speed = 0;
this.maxSpeed = this.game.road.segmentGap * 60;
this.accel = this.maxSpeed / 5;
this.breaking = -this.maxSpeed;
this.decel = -this.maxSpeed / 5;
this.offRoadDecel = -this.maxSpeed / 2;
this.offRoadMaxSpeed = this.maxSpeed / 4;
this.dx = this.game.road.width / 1000 * this.game.resolution.x / 2;
this._imgs = {width : 80, height : 41};
this._imgs.screenW = this.game.resolution.x / (this.game.road.lanes + 1);
this._imgs.screenH = this._imgs.screenW / this._imgs.width * this._imgs.height;
};
Car.prototype = {
loadImage : function() {
this.game.engine.load.image("player-left", "imgs/player_left.png");
this.game.engine.load.image("player-right", "imgs/player_right.png");
this.game.engine.load.image("player-straight", "imgs/player_straight.png");
},
init : function() {
this._imgs.straight = this.game.engine.cache.getImage('player-straight');
this._imgs.straightLeft = this.game.engine.cache.getImage('player-left');
this._imgs.straightRight = this.game.engine.cache.getImage('player-right');
this.bitmap = this.game.engine.add.bitmapData(
this._imgs.screenW,
this._imgs.screenH
);
this.game.engine.add.image(
(this.game.resolution.x - this._imgs.screenW) / 2,
(this.game.resolution.y - this._imgs.screenH),
this.bitmap
);
},
_increaseSpeed : function(increment) {
var speed = this.speed + increment;
if(speed < 0) speed = 0;
if(speed > this.maxSpeed) speed = this.maxSpeed;
this.speed = speed;
return this.speed;
},
isOffRoad : function() {
return this.offsetX > this.game.road.width / 2 ||
this.offsetX < -this.game.road.width / 2;
},
accelerate : function(t) {
if(this.isOffRoad() && this.speed > this.offRoadMaxSpeed) {
this._increaseSpeed(this.offRoadDecel * t);
} else {
this._increaseSpeed(this.accel * t);
}
return this.speed;
},
brake : function(t) {
return this._increaseSpeed(this.breaking * t);
},
decelerate : function(t) {
if(this.isOffRoad() && this.speed > this.offRoadMaxSpeed) {
this._increaseSpeed(this.offRoadDecel * t);
} else {
this._increaseSpeed(this.decel * t);
}
return this.speed;
},
turnRight : function(t) {
this.offsetX += this.dx * t * this.speed / this.maxSpeed;
if(this.offsetX > this.game.road.width) this.offsetX = this.game.road.width;
return this.offsetX;
},
turnLeft : function(t) {
this.offsetX -= this.dx * t * this.speed / this.maxSpeed;
if(this.offsetX < -this.game.road.width) this.offsetX = -this.game.road.width;
return this.offsetX;
},
run : function(t) {
this.mileage += this.speed * t;
if(this.mileage >= this.game.road.trackDistance) {
this.mileage -= this.game.road.trackDistance;
}
var percent = ((this.mileage + this.z) % this.game.road.segmentGap) / this.game.road.segmentGap,
segment = this.game.road.findSegment(this.mileage + this.z);
this.offsetY = segment.p1.world.y + (segment.p2.world.y - segment.p1.world.y) * percent;
return this.mileage;
},
render : function() {
var img;
if(this.game.key.left.isDown) {
img = this._imgs.straightLeft;
} else if(this.game.key.right.isDown) {
img = this._imgs.straightRight;
} else {
img = this._imgs.straight;
}
this.bitmap.ctx.clearRect(0, 0, this.bitmap.width, this.bitmap.height);
var bounce = 0;
if(this.speed > 0) {
bounce = (2 * Math.random() * this.speed / this.maxSpeed * this.game.resolution.y / 480) * 1;
}
this.bitmap.ctx.drawImage(img,
0, 0, this._imgs.width, this._imgs.height,
0, bounce, this._imgs.screenW, this._imgs.screenH);
this.bitmap.dirty = true;
}
};<file_sep>/js/road.js
var Road = function(game) {
this.game = game;
// 道路宽度(3d world)
this.width = 4000;
// 道路分段
this.segments = [];
// 道路分段数量
// this.segments.length = 500;
// 道路分段间距
this.segmentGap = 200;
// 总长度
// this.trackDistance = this.segments.length * this.segmentGap;
// 每3条分段使用相同配色
this.stripLength = 3;
// 车道数量
this.lanes = 3;
// 道路颜色
this.colors = {
light : {road : '#6B6B6B', grass : '#10AA10', rumble : '#555555', lane : '#CCCCCC'},
dark : {road : '#696969', grass : '#009A00', rumble : '#BBBBBB'}
};
this.setRoad();
};
Road.prototype = {
init : function() {
this.bitmap = this.game.engine.add.bitmapData(
this.game.resolution.x,
this.game.resolution.y
);
this.game.engine.add.image(0, 0, this.bitmap);
},
render : function() {
this.bitmap.ctx.clearRect(0, 0, this.game.resolution.x, this.game.resolution.y);
var base = this.findSegmentIndex(this.game.pseudo3DCamera.z + this.game.car.mileage),
maxy = this.game.resolution.y,
pseudo3DCamera = this.game.pseudo3DCamera,
car = this.game.car;
for(var i = 0; i < this.segments.length; i++) {
var cur = (base + i) % this.segments.length,
segment = this.segments[cur],
p1 = segment.p1,
p2 = segment.p2,
looped = cur < base;
this.project(p1, pseudo3DCamera.x + car.offsetX, pseudo3DCamera.y + car.offsetY,
pseudo3DCamera.z + (looped ? car.mileage - this.trackDistance : car.mileage));
this.project(p2, pseudo3DCamera.x + car.offsetX, pseudo3DCamera.y + car.offsetY,
pseudo3DCamera.z + (looped ? car.mileage - this.trackDistance : car.mileage));
if(p2.screen.y >= maxy) continue;
var rw1 = this.rumbleWidth(p1.screen.w),
rw2 = this.rumbleWidth(p2.screen.w);
//草地
this.polygon(this.bitmap.ctx,
0, p2.screen.y,
this.game.resolution.x, p2.screen.y,
this.game.resolution.x, p1.screen.y,
0, p1.screen.y,
segment.color.grass
);
//公路左边界
this.polygon(this.bitmap.ctx,
p1.screen.x - p1.screen.w / 2 - rw1, p1.screen.y,
p1.screen.x - p1.screen.w / 2, p1.screen.y,
p2.screen.x - p2.screen.w / 2, p2.screen.y,
p2.screen.x - p2.screen.w / 2 - rw2, p2.screen.y,
segment.color.rumble
);
//公路右边界
this.polygon(this.bitmap.ctx,
p1.screen.x + p1.screen.w / 2 + rw1, p1.screen.y,
p1.screen.x + p1.screen.w / 2, p1.screen.y,
p2.screen.x + p2.screen.w / 2, p2.screen.y,
p2.screen.x + p2.screen.w / 2 + rw2, p2.screen.y,
segment.color.rumble
);
//公路
this.polygon(this.bitmap.ctx,
p1.screen.x - p1.screen.w / 2, p1.screen.y,
p1.screen.x + p1.screen.w / 2, p1.screen.y,
p2.screen.x + p2.screen.w / 2, p2.screen.y,
p2.screen.x - p2.screen.w / 2, p2.screen.y,
segment.color.road
);
if(segment.color.lane) {
var lw1 = this.laneMarkerWidth(p1.screen.w),
lw2 = this.laneMarkerWidth(p2.screen.w);
for(var j = 1; j < this.lanes; j++) {
this.polygon(
this.bitmap.ctx,
p1.screen.x - p1.screen.w / 2 + p1.screen.w / this.lanes * j - lw1 / 2, p1.screen.y,
p1.screen.x - p1.screen.w / 2 + p1.screen.w / this.lanes * j + lw1 / 2, p1.screen.y,
p2.screen.x - p2.screen.w / 2 + p2.screen.w / this.lanes * j + lw2 / 2, p2.screen.y,
p2.screen.x - p2.screen.w / 2 + p2.screen.w / this.lanes * j - lw2 / 2, p2.screen.y,
segment.color.lane
);
}
}
maxy = p2.screen.y;
}
this.bitmap.dirty = true;
},
findSegmentIndex : function(z) {
return Math.floor(z / this.segmentGap) % this.segments.length;
},
findSegment : function(z) {
return this.segments[this.findSegmentIndex(z)];
},
rumbleWidth : function(projectedRoadWidth) {
return projectedRoadWidth / Math.max(6, 2 * this.lanes);
},
laneMarkerWidth: function(projectedRoadWidth) {
return projectedRoadWidth / Math.max(32, 8 * this.lanes);
},
polygon : function(ctx, x1, y1, x2, y2, x3, y3, x4, y4, color) {
ctx.fillStyle = color;
ctx.beginPath();
ctx.moveTo(x1, y1);
ctx.lineTo(x2, y2);
ctx.lineTo(x3, y3);
ctx.lineTo(x4, y4);
ctx.closePath();
ctx.fill();
},
project : function(p, cameraX, cameraY, cameraZ) {
p.camera = {
x : (p.world.x || 0) - cameraX,
y : (p.world.y || 0) - cameraY,
z : (p.world.z || 0) - cameraZ
};
var rate = this.game.cameraDepth / p.camera.z;
p.screen = {
x : Math.round(this.game.resolution.x / 2 + rate * p.camera.x),
y : Math.round(this.game.resolution.y / 2 - rate * p.camera.y),
w : Math.round(rate * this.width)
};
},
setRoad : function() {
// 初始化道路分段
var num = Road.LENGTH.SHORT;
var height = Road.HILL.LOW;
this.addRoad(num, num, num, 0, height/2);
this.addRoad(num, num, num, 0, -height);
this.addRoad(num, num, num, 0, height);
this.addRoad(num, num, num, 0, 0);
this.addRoad(num, num, num, 0, height/2);
this.addRoad(num, num, num, 0, 0);
this.addRoad(num, num, num, 0, -this._lastY() / this.segmentGap);
this.trackDistance = this.segments.length * this.segmentGap;
},
addSegment : function(curve, y) {
var i = this.segments.length,
lastY = this._lastY();
this.segments.push({
p1 : {world : {y : lastY, z : this.segmentGap * i}, camera : null, screen : null},
p2 : {world : {y : y, z : this.segmentGap * (i + 1)}, camera : null, screen : null},
color : Math.floor(i / this.stripLength) % 2 ? this.colors.dark : this.colors.light,
curve : curve
});
},
addRoad : function(enter, hold, leave, curve, y) {
var startY = this._lastY(),
endY = startY + y * this.segmentGap,
total = enter + hold + leave,
i;
for(i = 0; i < enter; i++) {
this.addSegment(Road.easeIn(0, curve, i / enter), Road.easeInOut(startY, endY, i / total));
}
for(i = 0; i < hold; i++) {
this.addSegment(curve, Road.easeInOut(startY, endY, (enter + i) / total));
}
for(i = 0; i < leave; i++) {
this.addSegment(Road.easeInOut(curve, 0, i / leave), Road.easeInOut(startY, endY, (enter + hold + i) / total));
}
},
_lastY : function() {
var i = this.segments.length;
return i === 0 ? 0 : this.segments[i - 1].p2.world.y;
}
};
Road.LENGTH = {NONE : 0, SHORT : 25, MEDIUM : 50, LONG : 100};
Road.HILL = {NONE : 0, LOW : 20, MEDIUM : 40, HIGH : 60};
Road.CURVE = {NONE : 0, EASY : 2, MEDIUM : 4, HARD : 6};
Road.easeInOut = function(a, b, percent) {
return a + (b - a) * ((-Math.cos(percent * Math.PI) / 2) + 0.5);
};
Road.easeIn = function(a, b, percent) {
return a + (b - a) * Math.pow(percent, 2);
};<file_sep>/host.js
var connect = require('connect'),
morgan = require('morgan'),
serveStatic = require('serve-static'),
nPath = require('path'),
app = connect(),
staticDir = nPath.join(process.cwd(), process.argv[2]);
app.use(morgan()).use(serveStatic(staticDir)).listen(80);
console.log("host " + staticDir + " at 127.0.0.1:80"); | 33bdb5801654b3aaff8a07238eb55478fdc4e080 | [
"JavaScript"
] | 3 | JavaScript | revcozmo/racer | 0007f49eedc3768d0f92b1057c0d83c7efc7257c | cc673b793a84bf60fddbb433b535ed013227418b | |
refs/heads/main | <file_sep>import React from 'react';
import { connect } from 'react-redux';
import publicacionesReducer from '../../reducers/publicacionesReducer';
import publicacionesAction from '../Publicaciones';
const Tabla = (props) => {
const ponerFilas = () => props.publicaciones.map((publicacion) => (
<tr key={publicacion.id}>
<td>
{ publicacion.title }
</td>
<td>
{ publicacion.body }
</td>
</tr>
));
return (
<div>
<table className="tabla">
<thead>
<tr>
<th>Título</th>
<th>Descripción</th>
</tr>
</thead>
<tbody>{ponerFilas()}</tbody>
</table>
</div>
)
}
const mapStateToProps = (reducers) => {
return reducers.publicacionesReducer;
}
export default connect(mapStateToProps, publicacionesAction) (Tabla);
<file_sep>import React from 'react';
import { connect } from 'react-redux';
import Spinner from '../General/Spinner';
import Fatal from '../General/Fatal';
const Comentarios = (props) => {
if(props.com_error && !props.comentarios.length){
return <Fatal mensaje={ props.com_error } />
}
if(props.com_cargando){
return <Spinner/>
}
const ponerComentarios = () => (
props.comentarios.map((comentario) => (
<li>
<b>
<u>
{ comentario.email }
</u>
</b>
<br/>
{ comentario.body }
</li>
))
);
return (
<ul>
{ ponerComentarios() }
</ul>
);
}
const mapStateToProps = ({publicacionesReducer}) => publicacionesReducer;
export default connect(mapStateToProps) (Comentarios);<file_sep>export const TRAER_TODAS = "tareas_traer_todos";
export const CARGANDO = "tareas_cargando";
export const ERROR = "tareas_error";
export const CAMBIO_USUARIO_ID = "cambio_usuario_id";
export const CAMBIO_USUARIO_TITULO = "cambio_usuario_titulo";
export const GUARDAR_TAREA = "guardar_tarea";
export const ACTUALIZAR = "actualizar_tarea";
export const LIMPIAR = "limpiar_tarea";
<file_sep>export const TRAER_TODOS = "usuarios_traer_todos";
export const CARGANDO = "usuarios_cargando";
export const ERROR = "usuarios_error";<file_sep>export const ACTUALIZAR = "publicaciones_actualizar";
export const CARGANDO = "publicaciones_cargando";
export const ERROR = "publicaciones_error";
export const COM_ACTUALIZAR = "comentarios_actualizar";
export const COM_CARGANDO = "comentarios_cargando";
export const COM_ERROR = "comentarios_error";<file_sep>import axios from 'axios';
import {
CARGANDO,
ERROR,
ACTUALIZAR,
COM_ACTUALIZAR,
COM_ERROR,
COM_CARGANDO,
} from '../types/publicacionesTypes';
import * as usuariosTypes from '../types/usuariosTypes';
const { TRAER_TODOS: USUARIOS_TRAER_TODOS } = usuariosTypes;
export const traerPorUsuario = (key) => async (dispatch, getState) => {
dispatch({
type: CARGANDO,
});
let { usuarios } = getState().usuariosReducer;
const { publicaciones } = getState().publicacionesReducer;
const usuario_id = usuarios[key].id;
try{
const respuesta = await axios.get(`https://jsonplaceholder.typicode.com/posts?userId=${usuario_id}`);
const nuevas = respuesta.data.map((publicacion) => ({
...publicacion,
comentarios: [],
abierto: false,
}));
const publicaciones_actualizadas = [
...publicaciones,
nuevas,
];
dispatch({
type: ACTUALIZAR,
payload: publicaciones_actualizadas
});
const publicaciones_key = publicaciones_actualizadas.length - 1;
const usuarios_actualizados = [...usuarios];
usuarios_actualizados[key] = {
...usuarios[key],
publicaciones_key
}
dispatch({
type: USUARIOS_TRAER_TODOS,
payload: usuarios_actualizados
});
}catch(error){
dispatch({
type:ERROR,
payload: 'Publicaciones no disponibles.'
});
}
};
export const abrirCerrar = (pub_key, com_key) => ((dispatch, getState) => {
const { publicaciones } = getState().publicacionesReducer;
const seleccionada = publicaciones[pub_key][com_key];
const actualizada = {
...seleccionada,
abierto: !seleccionada.abierto,
}
const publicaciones_actualizadas = [...publicaciones];
publicaciones_actualizadas[pub_key] = [
...publicaciones[pub_key]
]
publicaciones_actualizadas[pub_key][com_key] = actualizada;
dispatch({
type: ACTUALIZAR,
payload: publicaciones_actualizadas,
});
});
export const traerComentarios = (pub_key, com_key) => async (dispatch, getState) => {
dispatch({
type: COM_CARGANDO,
});
const { publicaciones } = getState().publicacionesReducer;
const seleccionada = publicaciones[pub_key][com_key];
try{
const respuesta = await axios.get(`https://jsonplaceholder.typicode.com/comments?postId=${seleccionada.id}`);
const actualizada = {
...seleccionada,
comentarios: respuesta.data,
};
const publicaciones_actualizadas = [...publicaciones];
publicaciones_actualizadas[pub_key] = [
...publicaciones[pub_key]
]
publicaciones_actualizadas[pub_key][com_key] = actualizada;
dispatch({
type: COM_ACTUALIZAR,
payload: publicaciones_actualizadas,
});
}catch(error){
dispatch({
type: COM_ERROR,
payload: 'Comentarios no disponibles.'
});
}
}; | cdd158286a4c293f8b14f208416dcddace8e09d5 | [
"JavaScript"
] | 6 | JavaScript | AlbertoZavala/platzi-blog | 033920859931a6e30ffc2bf52cc82b2c43c7d43b | 1ba8d42569e104ef78dfcab40a433e976454fa0e | |
refs/heads/master | <repo_name>cdpowell/test-project<file_sep>/mwFileStatusWebsite/__init__.py
from . import validator, constructor, compare
__version__ = '1.0.0'
<file_sep>/setup.py
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
import os
import sys
import re
from setuptools import setup, find_packages
if sys.argv[-1] == 'publish':
os.system('python3 setup.py sdist')
os.system('twine upload dist/*')
sys.exit()
def readme():
with open('README.md') as readme_file:
return readme_file.read()
def find_version():
with open('mwFileStatusWebsite/__init__.py', 'r') as fd:
version = re.search(r'^__version__\s*=\s*[\'"]([^\'"]*)[\'"]',
fd.read(), re.MULTILINE).group(1)
if not version:
raise RuntimeError('Cannot find version information')
return version
REQUIRES = [
"mwtab >= 1.2.4",
"pytest"
]
setup(
name='mwFileStatusWebsite',
version=find_version(),
author='<NAME>',
author_email='<EMAIL>',
description='Supplemental database for the Metabolomics Workbench data repository',
keywords='metabolomics workbench',
license='BSD',
packages=find_packages(),
platforms='any',
long_description=readme(),
install_requires=REQUIRES,
classifiers=[
'Development Status :: 4 - Beta',
'Environment :: Console',
'Intended Audience :: Developers',
'Intended Audience :: Science/Research',
'License :: OSI Approved :: BSD License',
'Operating System :: OS Independent',
'Programming Language :: Python :: 3.5',
'Programming Language :: Python :: 3.6',
'Programming Language :: Python :: 3.7',
'Programming Language :: Python :: 3.8',
'Programming Language :: Python :: 3.9',
'Programming Language :: Python :: 3.10',
'Topic :: Scientific/Engineering :: Bio-Informatics',
'Topic :: Software Development :: Libraries :: Python Modules',
],
)
<file_sep>/mwFileStatusWebsite/constructor.py
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
"""
constructor.py
~~~~~~~~~~~~~~~~
This script contains methods for generating HTML pages from validation dictionaries.
"""
import json
from datetime import datetime
import pkgutil
MESSAGE_COLOR = {
"Passing": "brightgreen",
"Validation Error": "orange",
"Parsing Error": "red",
"Missing/Blank": "lightgrey",
'Consistent': 'brightgreen',
'Inconsistent': 'orange',
'Not Checked': 'lightgrey'
}
MESSAGE_TO_LEVEL = {
"Passing": 0,
"Validation Error": 1,
"Parsing Error": 2,
"Missing/Blank": 3,
}
LEVEL_TO_MESSAGE = {
MESSAGE_TO_LEVEL[k]: k for k in MESSAGE_TO_LEVEL
}
# load in all the necessary HTML templates
INDEX_HEADER_TEMPLATE = pkgutil.get_data(__name__, 'templates/index_header_template.txt').decode('utf-8')
INDEX_TEMPLATE = pkgutil.get_data(__name__, 'templates/index_template.txt').decode('utf-8')
VAL_STATS_TEMPLATE = pkgutil.get_data(__name__, 'templates/statistics_template.txt').decode('utf-8')
COMP_STATS_TEMPLATE = pkgutil.get_data(__name__, 'templates/comparison_stats_template.txt').decode('utf-8')
HEADER_TEMPLATE = pkgutil.get_data(__name__, 'templates/header_template.txt').decode('utf-8')
GRID_TEMPLATE = pkgutil.get_data(__name__, 'templates/grid_template.txt').decode('utf-8')
GRID_ITEM_TEMPLATE = pkgutil.get_data(__name__, 'templates/grid_item_template.txt').decode('utf-8')
BADGE_TEMPLATE = pkgutil.get_data(__name__, 'templates/badge_template.txt').decode('utf-8')
DESC_TEMPLATE = "<div class=\"desc__grid__item\"{0}>{1}</div>"
def load_json(filepath):
"""Help function for loading in JSON data files.
:param filepath: Path to JSON file to be loaded.
:type filepath: str
:return: JSON dictionary object.
:rtype: dict
"""
with open(filepath, "r") as fh:
json_dict = json.loads(fh.read())
fh.close()
return json_dict
def generate_validation_stats_summary(validation_dict):
"""Method for generating the statistics to filling the HTML template with the current Metabolomics Workbench mwTab
files validation data.
:param validation_dict: Dictionary object containing the validation statuses for all validated mwTab data files.
:type validation_dict: dict
:return: Tuple containing the number of validated studies, number of validated analyses, and the dictionary
containing the validation data (eg. number Passing, number with Validation Errors, etc.).
:rtype: tuple
"""
num_studies = 0
num_analyses = 0
error_num_dict = {
key: {"txt": 0, "json": 0} for key in ["Passing", "Parsing Error", "Validation Error", "Missing/Blank"]
}
for study_id in validation_dict:
num_studies += 1
for analysis_id in validation_dict[study_id]["analyses"]:
num_analyses += 1
for file_format in ('txt', 'json'):
error_num_dict[validation_dict[study_id]["analyses"][analysis_id]["status"][file_format]][file_format] += 1
return num_studies, num_analyses, error_num_dict
def generate_comparison_stats_summary(validation_dict):
"""Method for generating the statistics to filling the HTML template with the current Metabolomics Workbench mwTab
files comparison data.
:param validation_dict: Dictionary object containing the validation statuses for all validated mwTab data files.
:type validation_dict: dict
:return: Tuple containing the number of validated studies, number of validated analyses, and the dictionary
containing the validation data (eg. number Passing, number with Validation Errors, etc.).
:rtype: tuple
"""
count_dict = {
'Consistent': 0,
'Inconsistent': 0,
'Not Checked': 0,
}
for study_id in validation_dict:
for analysis_id in validation_dict[study_id]['analyses']:
count_dict[validation_dict[study_id]['analyses'][analysis_id]['status']['comparison']] += 1
return count_dict['Consistent'], count_dict['Inconsistent'], count_dict['Not Checked']
def create_desc(params, tabs="\t"*6):
"""Method for parsing out STUDY or ANALYSIS description items from the generated validation nested dictionary and
returning a formatted string.
:param params: Nested validation dictionary or section of nested validation dictionary.
:type params: dict
:param tabs: White spacing for tabs.
:type tabs: str
:return: String containing formatted
:rtype: str
"""
desc_items = list()
for k in params:
desc_items.append(tabs + DESC_TEMPLATE.format("", k))
desc_items.append(tabs + DESC_TEMPLATE.format(" style=\"white-space:nowrap;overflow:hidden;text-overflow:ellipsis;width:calc(100%);\"", params.get(k)))
return "\n".join(desc_items)
def create_html(validation_dict, config_dict, output_filename):
"""Creates and saves HTML file based on given validation and config dictionaries.
:param validation_dict: Structured dictionary containing analyses statuses and other study information.
:type validation_dict: dict
:param config_dict: Dictionary containing GitHub repository information.
:type config_dict: dict
:param output_filename: Filename of HTML file to be created.
:type output_filename: str
:return: None
"""
with open(output_filename, "w") as fh:
#####################################
# write the HTML header information #
#####################################
fh.write(INDEX_HEADER_TEMPLATE.format(
config_dict['owner'],
config_dict['repo'],
str(datetime.now()),
))
#####################################################
# collect and write validation and comparison stats #
#####################################################
# collect general statistics for the run (number of available studies and analyses).
num_studies, num_analyses, error_dict = generate_validation_stats_summary(validation_dict)
# Fill out the statistics_template and comparison_stats_template.
num_errors = list()
for error_type in error_dict:
for file_format in error_dict[error_type]:
num_errors.append(error_dict[error_type][file_format])
# writes the validation and comparison stats sections to the HTML file
fh.write(VAL_STATS_TEMPLATE.format(num_studies, num_analyses, *num_errors, config_dict['owner'], config_dict['repo']))
fh.write(COMP_STATS_TEMPLATE.format(*generate_comparison_stats_summary(validation_dict)))
################################
# generate file status section #
################################
# file_status_list = []
for study_id in validation_dict:
# # Add space between grid items
# if file_status_list:
# file_status_list.append()
# Add study header
# Adds header line (grid)
# Adds study meta data
height = 1*len(validation_dict[study_id]["params"])
fh.write(HEADER_TEMPLATE.format(
study_id,
validation_dict[study_id]["params"].get("STUDY_TITLE"),
validation_dict[study_id]["params"].get("INSTITUTE"),
validation_dict[study_id]["params"].get("LAST_NAME"),
validation_dict[study_id]["params"].get("FIRST_NAME"),
" style=\"height:" + str(height) + "em;max-height:" + str(height) + "\"",
create_desc(validation_dict[study_id]["params"])
))
grid_item_list = []
for analysis_id in validation_dict[study_id]["analyses"]:
badge_list = []
for format_type in validation_dict[study_id]["analyses"][analysis_id]["status"]:
badge_list.append(BADGE_TEMPLATE.format(
analysis_id,
format_type,
MESSAGE_COLOR[validation_dict[study_id]["analyses"][analysis_id]["status"][format_type]],
validation_dict[study_id]["analyses"][analysis_id]["status"][format_type],
config_dict['owner'],
config_dict['repo']
))
# adds the colored analysis button
grid_item_list.append(GRID_ITEM_TEMPLATE.format(
analysis_id,
MESSAGE_COLOR[LEVEL_TO_MESSAGE[max([
MESSAGE_TO_LEVEL[value] for value in validation_dict[study_id]["analyses"][analysis_id]["status"].values() if value in MESSAGE_TO_LEVEL.keys()
])]],
"\n".join(badge_list),
create_desc(validation_dict[study_id]["analyses"][analysis_id]["params"])
))
fh.write(GRID_TEMPLATE.format("\n".join(grid_item_list)))
fh.write("\t\t\t<br>")
# close file
fh.write("\t\t</div>\n\t</body>\n</html>\n")
def create_error_dicts(validation_dict, status_str, file_format=None):
"""Method for creating a dictionary containing the validation status and additional parameters of analyses with
indicated validation status.
:param validation_dict: Structured dictionary containing analyses statuses and other study information.
:type validation_dict: dict
:param status_str: Analysis validation status to be searched for.
:type status_str: str
:param file_format: Indicates which file format to be searched (if only one).
:type file_format: str or bool
:return: Structured dictionary containing analyses statuses and other study information for analyses with indicated
validation status.
:rtype: dict
"""
status_dict = dict()
for study_id in validation_dict:
for analysis_id in validation_dict[study_id]["analyses"]:
# both file formats have the same validation status
if not file_format:
file_format_status_set = {validation_dict[study_id]["analyses"][analysis_id]["status"]['json'],
validation_dict[study_id]["analyses"][analysis_id]["status"]['txt']}
if {status_str} == file_format_status_set:
status_dict.setdefault(study_id, dict()).setdefault("params", validation_dict[study_id]["params"])
status_dict[study_id].setdefault("analyses", dict())[analysis_id] = \
validation_dict[study_id]["analyses"][analysis_id]
# TODO: Allow specifying which file format to be checked
# only one file format has a given status
else:
if status_str in set(validation_dict[study_id]["analyses"][analysis_id]["status"].values()):
status_dict.setdefault(study_id, dict()).setdefault("params", validation_dict[study_id]["params"])
status_dict[study_id].setdefault("analyses", dict())[analysis_id] = \
validation_dict[study_id]["analyses"][analysis_id]
return status_dict
<file_sep>/mwFileStatusWebsite/validator.py
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
"""
validator.py
~~~~~~~~~~~~
This script contains methods for validating all available mwTab data files, creating log files, and generating a JSON
file containing the validation metadata.
"""
import mwFileStatusWebsite.compare
import mwtab
import json
import re
from datetime import datetime
from os import walk
from os.path import join, isdir, isfile
from time import sleep
MW_REST_URL = "https://www.metabolomicsworkbench.org/rest/study/analysis_id/{}/mwtab/{}"
SLEEP_TIME = 1
NUM_TRIES = 3
def retrieve_mwtab_files(verbose=False):
"""Method for retrieving a dictionary of Metabolomics Workbench file identifiers.
Example:
{
'ST000001': [
'AN000001',
...
],
...
}
:param bool verbose: Run in verbose mode.
:return: Dictionary of study IDs (keys) and their associated lists of analysis IDs (values).
"""
try:
study_analysis_dict = mwtab.mwrest._pull_study_analysis()
# count the number of present studies and analyses
num_studies = len(study_analysis_dict)
num_analyses = sum([len(study_analysis_dict[k]) for k in study_analysis_dict])
if verbose:
print("{} studies found".format(num_studies))
print("{} analyses found".format(num_analyses))
return study_analysis_dict
# cannot access Metabolomics Workbench REST server
except Exception as e:
print("Unable to access https://www.metabolomicsworkbench.org/")
print(e)
exit()
def create_validation_dict(study_analysis_dict):
"""Method for creating a null structured validation dictionary.
:param study_analysis_dict: Dictionary of Metabolomics study IDs (key) and lists of their associated analysis IDs
(value).
:type study_analysis_dict: dict
:return: Returns a structured dictionary to contain analysis validation statuses and associated study data.
:rtype: dict
"""
# setup some variables for collecting validation statuses
validation_dict = {
study_id: {
"params": {}, # TODO:Change to STUDY_params
"analyses": {
analysis_id: {
"params": {
"ANALYSIS_ID": analysis_id
},
"status": {
"txt": None,
"json": None
}
} for analysis_id in sorted(study_analysis_dict[study_id])
}
} for study_id in sorted(study_analysis_dict.keys())
}
return validation_dict
def _validate(validation_dict, study_id, analysis_id, file_format, save_path=None):
"""Helper function for performing validation of a specified mwTab data file given the files; study ID, analysis ID,
and file format (.txt or .json).
:param validation_dict: Structured dictionary containing analyses statuses and other study information.
:type validation_dict: dict
:param study_id: Metabolomics Workbench study ID string (eg. ST000001).
:type study_id: str
:param analysis_id: Metabolomics Workbench analysis ID string (eg. AN000001).
:type analysis_id: str
:param file_format: File format extension string (either: 'txt' or 'json').
:type file_format: str
:param save_path: Boolean value indicating if retrieved analyses should be save.
:type save_path: bool or str
:return: Tuple containing of the validated mwtab file object and the string validation log.
:rtype: tuple
"""
mwtabfile = next(mwtab.read_files(MW_REST_URL.format(analysis_id, file_format)))
# allows saving out the retrieved non-validated mwTab analysis files.
if save_path:
with open(join(save_path, analysis_id + '.' + file_format), 'w') as fh:
mwtabfile.write(fh, 'mwtab' if file_format == 'txt' else 'json')
sleep(SLEEP_TIME)
# TODO: Allow validating METABOLITES section
validated_mwtabfile, validation_log = mwtab.validate_file(mwtabfile, metabolites=False)
# parse validation status (e.g. "Passing") from validation log
status_str = re.search(r'Status.*', validation_log).group(0).split(': ')[1]
if status_str == 'Passing':
validation_dict[study_id]["analyses"][analysis_id]["status"][file_format] = "Passing"
elif status_str == 'Contains Validation Errors':
validation_dict[study_id]["analyses"][analysis_id]["status"][file_format] = "Validation Error"
# parse out STUDY block parameters
if not validation_dict[study_id]["params"]:
validation_dict[study_id]["params"] = mwtabfile["STUDY"]
return validated_mwtabfile, validation_log
def validate(validation_dict, study_id, analysis_id, file_format, save_path=None):
"""Method for validating a given Metabolomics Workbench mwTab file.
Creates a validation log and adds validation status to the given validation_dict dictionary. Fetches files using the
``mwtab`` Python3 packages built in methods for accessing Metabolomics Workbench's REST API. Performs three attempts
to retrieve a file before labeling it as "Missing/Blank".
:param validation_dict: Structured dictionary containing analyses statuses and other study information.
:type validation_dict: dict
:param study_id: Metabolomics Workbench study ID string (eg. ST000001).
:type study_id: str
:param analysis_id: Metabolomics Workbench analysis ID string (eg. AN000001).
:type analysis_id: str
:param file_format: File format extension string (either: 'txt' or 'json').
:type file_format: str
:param save_path: Boolean value indicating if retrieved analyses should be save.
:type save_path: bool or str
:return: Tuple containing of the validated mwtab file object and the string validation log.
:rtype: tuple
"""
error = False
try:
validated_mwtabfile, validation_log = _validate(validation_dict, study_id, analysis_id, file_format, save_path)
except Exception as e:
# error is one of; 1) temporary server error, 2) source is blank, or 3) source cannot be parsed
# check to see if temporary server error
error = True
for x in range(NUM_TRIES): # try three times to see if there is a temporary server error
try:
validated_mwtabfile, validation_log = _validate(validation_dict, study_id, analysis_id, file_format)
error = False
break
except Exception:
pass
# not temporary server error, either source is blank or source cannot be parsed
if error:
# blank source given
if type(e) == ValueError and e.args[0] == "Blank input string retrieved from source.":
validation_dict[study_id]["analyses"][analysis_id]["status"][file_format] = "Missing/Blank"
else:
validation_dict[study_id]["analyses"][analysis_id]["status"][file_format] = "Parsing Error"
# create validation log for missing or unparsable files.
validation_log = mwtab.validator.VALIDATION_LOG_HEADER.format(
str(datetime.now()),
mwtab.__version__,
MW_REST_URL.format(analysis_id, file_format),
study_id,
analysis_id,
file_format
)
validation_log += \
"\nStatus:" + \
validation_dict[study_id]["analyses"][analysis_id]["status"][file_format] + \
"\n" + str(e)
if not error:
return validated_mwtabfile, validation_log
else:
return {}, validation_log
def validate_mwtab_rest(input_dict=None, logs_path='docs/validation_logs', output_file="tmp.json",
verbose=False, save_path=None):
"""Method for validating all available Metabolomics Workbench mwTab formatted data files.
:param input_dict: Dictionary of Metabolomics study IDs (key) and lists of their associated analysis IDs (value).
:type input_dict: dict
:param logs_path: File path to the directory validation logs are to be saved to.
:type logs_path: str
:param output_file: File path for the structured dictionary containing analyses statuses and other study information
to be saved to.
:type output_file: str
:param verbose: Run in verbose mode.
:type verbose: bool
:param save_path: Directory path for retrieved Metabolomics Workbench analysis data files to be saved in.
:type save_path: str
:return: Structured dictionary containing analyses statuses and other study information.
:rtype: dict
"""
if verbose:
print("Running mwTab file validation.")
print("\tmwtab version:", mwtab.__version__)
# collect dictionary of studies and their analyses
if input_dict:
study_analysis_dict = input_dict
else:
study_analysis_dict = retrieve_mwtab_files(verbose)
# create the validation dict
validation_dict = create_validation_dict(study_analysis_dict)
for study_id in sorted(study_analysis_dict.keys()):
if verbose:
print("Validating study:", study_id)
for analysis_id in study_analysis_dict[study_id]:
if verbose:
print("\t", analysis_id)
# retrieve file in both its 'txt' and 'json' formats
txt_mwtab_file, txt_validation_log = validate(validation_dict, study_id, analysis_id, 'txt', save_path=save_path)
json_mwtab_file, json_validation_log = validate(validation_dict, study_id, analysis_id, 'json', save_path=save_path)
# if both formats are available and parsable, compare the two files
validation_dict[study_id]["analyses"][analysis_id]["status"]['comparison'] = 'Not Checked'
if txt_mwtab_file and json_mwtab_file: # both files passed validation and can be compared
comparison_list = mwFileStatusWebsite.compare.compare(txt_mwtab_file, json_mwtab_file)
if comparison_list:
comparison_status = 'Inconsistent'
error_str = '\n'.join([str(error) for error in comparison_list])
else:
comparison_status = 'Consistent'
error_str = ''
validation_dict[study_id]["analyses"][analysis_id]["status"]['comparison'] = comparison_status
comparison_log = mwFileStatusWebsite.compare.COMPARISON_LOG.format(
str(datetime.now()),
mwtab.__version__,
MW_REST_URL.format(analysis_id, '...'),
study_id,
analysis_id,
comparison_status
) + error_str
with open(join(logs_path, '{}_comparison.log').format(analysis_id), 'w') as fh:
fh.write(comparison_log)
# save out each files validation log
with open(join(logs_path, '{}_{}.log'.format(analysis_id, 'txt')), 'w') as fh:
fh.write(txt_validation_log)
with open(join(logs_path, '{}_{}.log'.format(analysis_id, 'json')), 'w') as fh:
fh.write(json_validation_log)
# export validation status dictionary
with open(output_file, "w") as fh:
fh.write(json.dumps(validation_dict, indent=4))
return validation_dict
def validate_mwtab_files(file_path=None, logs_path='docs/validation_logs', output_file="tmp.json", verbose=False,):
"""Validate and create a validation dictionary for a single Metabolomics Workbench analysis data file (mwTab or
JSON) or a directory of data files.
:param file_path: File path to directory containing Metabolomics Workbench analysis data files to be validated.
:type file_path: str
:param logs_path: File path to the directory validation logs are to be saved to.
:type logs_path: str
:param output_file: File path for the structured dictionary containing analyses statuses and other study information
to be saved to.
:type output_file: str
:param verbose: Run in verbose mode.
:type verbose: bool
:return: Structured dictionary containing analyses statuses and other study information.
:rtype: dict
"""
# check to see if a directory or file was passed in
if isdir(file_path):
_, _, filenames = next(walk(file_path))
analysis_ids = sorted({filename.split('.')[0] for filename in filenames})
elif isfile(file_path):
analysis_ids = []
else:
raise ValueError("'file_path' is not a valid directory or file.")
validation_dict = dict()
for analysis_id in analysis_ids:
pass
<file_sep>/mwFileStatusWebsite/cli.py
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
"""
The mwFileStatusWebsite command-line interface
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Usage:
mwFileStatusWebsite -h | --help
mwFileStatusWebsite --version
mwFileStatusWebsite validate [--to-path=<path>]
mwFileStatusWebsite generate
Options:
-h, --help Show this screen.
--version Show version.
--verbose Enable verbose processing.
--to-path=<path> Path to directory for retrieved analyses to be saved in.
"""
from . import validator, constructor
def cli(cmdargs):
if cmdargs['validate']:
validator.validate_mwtab_rest(save_path=cmdargs['--to-path'])
elif cmdargs['generate']:
# create the main webpage (index.html)
validation_dict = constructor.load_json('tmp.json')
config_dict = constructor.load_json('docs/config.json')
constructor.create_html(validation_dict, config_dict, 'index.html')
# create the passing.html page
# contains only analyses which one or both formats (mwTab and JSON) are passing
passing = constructor.create_error_dicts(validation_dict, 'Passing', True)
constructor.create_html(passing, config_dict, 'passing.html')
# create the validation_error.html page
# contains analyses which both formats (mwTab and JSON) have validation errors.
validation = constructor.create_error_dicts(validation_dict, 'Validation Error')
constructor.create_html(validation, config_dict, 'validation_error.html')
# create the parsing_error.html page
# contains analyses which both formats (mwTab and JSON) have parsing errors.
parsing = constructor.create_error_dicts(validation_dict, 'Parsing Error')
constructor.create_html(parsing, config_dict, 'parsing_error.html')
# create the missing.html page
# contains analyses which both formats (mwTab and JSON) are missing.
missing = constructor.create_error_dicts(validation_dict, 'Missing/Blank')
constructor.create_html(missing, config_dict, 'missing.html')
<file_sep>/tests/test_compare.py
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
"""
test_compare.py
~~~~~~~~~~~~~~~
This script contains methods comparing two Metabolomics Workbench file in `~mwtab.mwtab.MWTabFile` object and assert
that the files (objects) are the same.
"""
import pytest
import mwFileStatusWebsite
import mwtab
from urllib.request import urlopen
from time import sleep
import pkgutil
from collections import OrderedDict
@pytest.mark.parametrize('mwtabfile_1, mwtabfile_2, error', [
({"a": {"b": 1}}, {"a": {"b": 1}}, False), # same blocks, same items
({"a": {"b": 1}}, {"a": {"c": 1}}, False), # same blocks, different item blocks
({"a": {"b": 1}}, {"b": {"b": 1}}, True), # different blocks
({"a": {"b": 1}}, {"a": {"b": 1}, "c": {"b": 1}}, True), # one file has an additional block
({"a": {"b": 1}}, {}, True), # one file is null
])
def test_compare_blocks(mwtabfile_1, mwtabfile_2, error):
try:
mwFileStatusWebsite.compare.compare_blocks(mwtabfile_1, mwtabfile_2)
if error: # test should have raise an error
assert False
except Exception as e:
if not error:
assert False
@pytest.mark.parametrize('mwtabfile_1, mwtabfile_2, error', [
({"PROJECT": {"b": 1}}, {"PROJECT": {"b": 1}}, False), # same blocks, same items
({"PROJECT": {"b": 1}}, {"PROJECT": {"c": 1}}, True), # same blocks, different item blocks
({"PROJECT": {"b": 1}}, {"PROJECT": {}}, True), # same blocks, one block is null
({"PROJECT": {"b": 1}}, {"PROJECT": {"b": 1}, "STUDY": {"a": 1}}, False), # different blocks, same items in mutual blocks
])
def test_compare_block_items(mwtabfile_1, mwtabfile_2, error):
errors = mwFileStatusWebsite.compare.compare_block_items(mwtabfile_1, mwtabfile_2)
if bool(errors) != error:
assert False
@pytest.mark.parametrize('mwtabfile_1, mwtabfile_2, error', [
# same SSF section
(
{'SUBJECT_SAMPLE_FACTORS':[
OrderedDict([('Subject ID', '-'), ('Sample ID', '1'), ('Factors', {'Factor1': 'Blah'})])
]},
{'SUBJECT_SAMPLE_FACTORS': [
OrderedDict([('Subject ID', '-'), ('Sample ID', '1'), ('Factors', {'Factor1': 'Blah'})])
]},
False
),
# different 'Sample ID'
(
{'SUBJECT_SAMPLE_FACTORS': [
OrderedDict([('Subject ID', '-'), ('Sample ID', '1'), ('Factors', {'Factor1': 'Blah'})])
]},
{'SUBJECT_SAMPLE_FACTORS': [
OrderedDict([('Subject ID', '-'), ('Sample ID', '2'), ('Factors', {'Factor1': 'Blah'})])
]},
True
),
# different factor values
(
{'SUBJECT_SAMPLE_FACTORS': [
OrderedDict([('Subject ID', '-'), ('Sample ID', '1'), ('Factors', {'Factor1': 'Blah'})])
]},
{'SUBJECT_SAMPLE_FACTORS': [
OrderedDict([('Subject ID', '-'), ('Sample ID', '1'), ('Factors', {'Factor1': 'Meh'})])
]},
True
)
])
def test_compare_block_items(mwtabfile_1, mwtabfile_2, error):
try:
mwFileStatusWebsite.compare.compare_subject_sample_factors(mwtabfile_1, mwtabfile_2)
if error: # test should have raise an error
assert False
except Exception as e:
if not error:
assert False
@pytest.mark.parametrize('mwtabfile_1, mwtabfile_2, error', [
# same "_DATA" section
(
{'MS_METABOLITE_DATA': {
'Units': 'test',
'Data': [OrderedDict([('Metabolite', 'Test'), ('Subject_1', 1000)])],
'Metabolites': [OrderedDict([('Metabolite', 'Test'),('Value_1', 'Test')])],
}},
{'MS_METABOLITE_DATA': {
'Units': 'test',
'Data': [OrderedDict([('Metabolite', 'Test'), ('Subject_1', 1000)])],
'Metabolites': [OrderedDict([('Metabolite', 'Test'), ('Value_1', 'Test')])],
}},
False
),
# different "_DATA" sections
(
{'MS_METABOLITE_DATA': {
'Units': 'test',
'Data': [OrderedDict([('Metabolite', 'Test'), ('Subject_1', 1000)])],
'Metabolites': [OrderedDict([('Metabolite', 'Test'), ('Value_1', 'Test')])],
}},
{'MNMR_BINNED_DATA': {
'Units': 'test',
'Data': [OrderedDict([('Metabolite', 'Test'), ('Subject_1', 1000)])],
'Metabolites': [OrderedDict([('Metabolite', 'Test'), ('Value_1', 'Test')])],
}},
True
),
# different "Units" subsections
(
{'MS_METABOLITE_DATA': {
'Units': 'test',
'Data': [OrderedDict([('Metabolite', 'Test'), ('Subject_1', 1000)])],
'Metabolites': [OrderedDict([('Metabolite', 'Test'), ('Value_1', 'Test')])],
}},
{'MNMR_BINNED_DATA': {
'Units': 'test2',
'Data': [OrderedDict([('Metabolite', 'Test'), ('Subject_1', 1000)])],
'Metabolites': [OrderedDict([('Metabolite', 'Test'), ('Value_1', 'Test')])],
}},
True
),
# different "Data" sections
(
{'MS_METABOLITE_DATA': {
'Units': 'test',
'Data': [OrderedDict([('Metabolite', 'Test'), ('Subject_1', 1000)])],
'Metabolites': [OrderedDict([('Metabolite', 'Test'), ('Value_1', 'Test')])],
}},
{'MNMR_BINNED_DATA': {
'Units': 'test',
'Data': [OrderedDict([('Metabolite', 'Test2'), ('Subject_1', 1000)])],
'Metabolites': [OrderedDict([('Metabolite', 'Test'), ('Value_1', 'Test')])],
}},
True
),
# different "Metabolites" sections
(
{'MS_METABOLITE_DATA': {
'Units': 'test',
'Data': [OrderedDict([('Metabolite', 'Test'), ('Subject_1', 1000)])],
'Metabolites': [OrderedDict([('Metabolite', 'Test'), ('Value_1', 'Test')])],
}},
{'MNMR_BINNED_DATA': {
'Units': 'test',
'Data': [OrderedDict([('Metabolite', 'Test'), ('Subject_1', 1000)])],
'Metabolites': [OrderedDict([('Metabolite', 'Test2'), ('Value_1', 'Test')])],
}},
True
),
])
def test_compare_data(mwtabfile_1, mwtabfile_2, error):
errors = mwFileStatusWebsite.compare.compare_data(mwtabfile_1, mwtabfile_2)
if errors and not error:
assert False
if not errors and error:
assert False
<file_sep>/mwFileStatusWebsite/compare.py
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
"""
compare.py
~~~~~~~~~~
This script contains methods comparing two Metabolomics Workbench file in `~mwtab.mwtab.MWTabFile` object and assert
that the files (objects) are the same.
TODO: This should be migrated into the mwtab Python library as a ~mwtab.mwtab.MWTabFile.equals() functionality or
TODO: similar.
"""
import operator
ITEM_SECTIONS = {
# "METABOLOMICS WORKBENCH",
"PROJECT",
"STUDY",
"ANALYSIS",
"SUBJECT",
"COLLECTION",
"TREATMENT",
"SAMPLEPREP",
"CHROMATOGRAPHY",
"MS",
"NM"
}
DATA_BLOCKS = {
'MS_METABOLITE_DATA',
'NMR_BINNED_DATA',
'NMR_METABOLITE_DATA'
}
COMPARISON_LOG = \
"""Comparison Log
{}
mwtab Python Library Version: {}
Source: {}
Study ID: {}
Analysis ID: {}
Status: {}
"""
def compare_blocks(mwtabfile_1, mwtabfile_2):
"""Method for comparing the section headers of two Metabolomics Workbench file in `~mwtab.mwtab.MWTabFile` object
format. Raises AssertionError if false.
:param mwtabfile_1: First Metabolomics Workbench file in mwtab format to be compared.
:type mwtabfile_1: :py:class:`~mwtab.mwtab.MWTabFile`
:param mwtabfile_2: Second Metabolomics Workbench file in mwtab format to be compared.
:type mwtabfile_2: :py:class:`~mwtab.mwtab.MWTabFile`
:return: None
"""
if not mwtabfile_1.keys() == mwtabfile_2.keys():
raise AssertionError(
"mwTab files contain different blocks: \"{}\"".format(
mwtabfile_1.keys() ^ mwtabfile_2.keys() # symmetric difference
)
)
def compare_block_items(mwtabfile_1, mwtabfile_2):
"""Method for comparing the items (keys and values) present within the sections of two Metabolomics Workbench file
in `~mwtab.mwtab.MWTabFile` object format.
:param mwtabfile_1: First Metabolomics Workbench file in mwtab format to be compared.
:type mwtabfile_1: :py:class:`~mwtab.mwtab.MWTabFile`
:param mwtabfile_2: Second Metabolomics Workbench file in mwtab format to be compared.
:type mwtabfile_2: :py:class:`~mwtab.mwtab.MWTabFile`
:return: List of errors.
:rtype: list
"""
error_list = list()
for section_key in set(mwtabfile_1.keys()) & set(mwtabfile_2.keys()) & ITEM_SECTIONS:
try:
if mwtabfile_1[section_key].items() ^ mwtabfile_2[section_key].items():
error_list.append(
AssertionError(
"Sections \"{}\" contain missmatched items: {}".format(
section_key,
mwtabfile_1[section_key].items() ^ mwtabfile_2[section_key].items()
)
)
)
except Exception as e:
error_list.append(e)
return error_list
def compare_subject_sample_factors(mwtabfile_1, mwtabfile_2):
"""Method for comparing the items (keys and values) present within the sections of two Metabolomics Workbench file
in `~mwtab.mwtab.MWTabFile` object format.
:param mwtabfile_1: First Metabolomics Workbench file in mwtab format to be compared.
:type mwtabfile_1: :py:class:`~mwtab.mwtab.MWTabFile`
:param mwtabfile_2: Second Metabolomics Workbench file in mwtab format to be compared.
:type mwtabfile_2: :py:class:`~mwtab.mwtab.MWTabFile`
:return: None
"""
new_mwtabfile_1_SSF_list = sorted(mwtabfile_1['SUBJECT_SAMPLE_FACTORS'], key=operator.itemgetter('Sample ID'))
new_mwtabfile_2_SSF_list = sorted(mwtabfile_2['SUBJECT_SAMPLE_FACTORS'], key=operator.itemgetter('Sample ID'))
if new_mwtabfile_1_SSF_list != new_mwtabfile_2_SSF_list:
raise AssertionError("mwTab files contain different 'SUBJECT_SAMPLE_FACTORS' sections.")
def compare_data(mwtabfile_1, mwtabfile_2):
"""Method for comparing "_DATA" blocks present within two Metabolomics Workbench file in `~mwtab.mwtab.MWTabFile`
object format.
:param mwtabfile_1: First Metabolomics Workbench file in mwtab format to be compared.
:type mwtabfile_1: :py:class:`~mwtab.mwtab.MWTabFile`
:param mwtabfile_2: Second Metabolomics Workbench file in mwtab format to be compared.
:type mwtabfile_2: :py:class:`~mwtab.mwtab.MWTabFile`
:return: List of errors.
:rtype: list
"""
data_section = set(mwtabfile_1.keys()) & set(mwtabfile_2.keys()) & DATA_BLOCKS
# data block is not consistently present across files
if not data_section or type(data_section) != set:
return [AssertionError("Unable to find '_DATA' block in given files.")]
else:
data_section = list(data_section)[0]
error_list = list()
subsections = set(mwtabfile_1[data_section].keys()) & set(mwtabfile_2[data_section].keys())
if mwtabfile_1[data_section].keys() != mwtabfile_2[data_section].keys():
error_list.append(AssertionError("'_DATA' blocks do not contain the same subsections: {}".format(
set(mwtabfile_1[data_section].keys()) ^ set(mwtabfile_2[data_section].keys())
)))
# compare "Units"
if 'Units' in subsections:
if mwtabfile_1[data_section]['Units'] != mwtabfile_2[data_section]['Units']:
error_list.append(AssertionError("'Units' section of '{}' block do not match.".format(data_section)))
# compare "Metabolites"
if 'Metabolites' in subsections:
try:
new_mwtabfile_1_metabolites_list = sorted(mwtabfile_1[data_section]['Metabolites'],
key=operator.itemgetter('Metabolite'))
new_mwtabfile_2_metabolites_list = sorted(mwtabfile_2[data_section]['Metabolites'],
key=operator.itemgetter('Metabolite'))
if new_mwtabfile_1_metabolites_list != new_mwtabfile_2_metabolites_list:
error_list.append(AssertionError("'Metabolites' section of '{}' block do not match.".format(data_section)))
except Exception as e:
error_list.append(e)
# compare "Data"
if 'Data' in subsections:
try:
new_mwtabfile_1_data_list = sorted(mwtabfile_1[data_section]['Data'],
key=operator.itemgetter('Metabolite'))
new_mwtabfile_2_data_list = sorted(mwtabfile_2[data_section]['Data'],
key=operator.itemgetter('Metabolite'))
if new_mwtabfile_1_data_list != new_mwtabfile_2_data_list:
error_list.append(AssertionError("'Data' section of '{}' block do not match.".format(data_section)))
except Exception as e:
error_list.append(e)
return error_list
def compare(mwtabfile_1, mwtabfile_2):
"""Method for comparing two Metabolomics Workbench file in `~mwtab.mwtab.MWTabFile` object format to assert that
they are the same. Raises AssertionError if false.
:param mwtabfile_1: First Metabolomics Workbench file in mwtab format to be compared.
:type mwtabfile_1: :py:class:`~mwtab.mwtab.MWTabFile`
:param mwtabfile_2: Second Metabolomics Workbench file in mwtab format to be compared.
:type mwtabfile_2: :py:class:`~mwtab.mwtab.MWTabFile`
:return: Log of assertion errors.
"""
error_list = list()
# compare files to assert they have the same section headings.
try:
compare_blocks(mwtabfile_1, mwtabfile_2)
except Exception as e:
error_list.append(e)
# compare files to assert they have the same items in each appropriate block (e.g. PROJECT, STUDY, etc.).
error_list.extend(compare_block_items(mwtabfile_1, mwtabfile_2)) # no issue if compare_block_keys() returns a null list.
# check that the SUBJECT_SAMPLE_FACTORS sections match
try:
compare_subject_sample_factors(mwtabfile_1, mwtabfile_2)
except Exception as e:
error_list.append(e)
error_list.extend(compare_data(mwtabfile_1, mwtabfile_2))
return error_list
<file_sep>/mw_website.sh
#!/bin/bash
# A Shell Script to update the Metabolomics Workbench File Status website
# <NAME> - 2022/02/20
###########################
# change directory to tmp #
###########################
cd /tmp
###################
# start ssh agent #
###################
eval "$(ssh-agent -s)"
ssh-add /root/.ssh/id_ed25519_github
##############################
# clone mw file status repo #
##############################
git clone <EMAIL>:MoseleyBioinformaticsLab/mwFileStatusWebsite.git
cd mwFileStatusWebsite/
###################################################
# install virtualenv and create a new environment #
###################################################
python3 -m pip install virtualenv
python3 -m virtualenv venv
source venv/bin/activate
python3 -m pip install mwtab
python3 -m pip install -e ../mwFilesStatusWebsite
########################
# update website files #
########################
#python3 -m validator/validator.py
#python3 -m validator/constructor.py
python3 -m mwFileStatusWebsite validate
python3 -m mwFileStatusWebsite generate
###################
# start ssh agent #
###################
eval "$(ssh-agent -s)"
ssh-add /root/.ssh/id_ed25519_github
#############################
# add updated files to repo #
#############################
git add docs/validation_logs index.html missing.html parsing_error.html passing.html validation_error.html
now=$(date +'%Y/%m/%d')
git commit -m "Weekly update for $now"
git push
#####################
# remove everything #
#####################
cd ..
rm -rf mwFileStatusWebsite/
<file_sep>/README.md
# Metabolomics Workbench File Status Website
This repository holds the scripts required for creating
and updating the Metabolomics Workbench File Status
website along with the validation longs for each
available analysis in both `JSON` and `mwTab` file
formats.
## Usage
The Metabolomics Workbench File Status website can be
accessed through it's GitHub Pages hosted webpage at:
https://moseleybioinformaticslab.github.io/mwFileStatusWebsite/
Individual validation files can be accessed in the
[docs/validation_logs/](https://github.com/moseleybioinformaticslab/mwFileStatusWebsite/tree/master/docs/validation_logs)
directory in this repository.
## Installation
The `mwFilesStatus` website can be run locally but is intended to be setup on a Virtual Machine for automated updating.
### Basic Installation
Clone the repository
```bash
git clone <EMAIL>:MoseleyBioinformaticsLab/mwFileStatusWebsite.git
cd mwFileStatusWebsite
```
Create a virtual environment and install the `mwFileStatusWebsite` package locally through `pip`.
```python
python3 -m virtualenv venv
source venv/bin/activate
python3 -m pip install -e ../mwFileStatusWebsite
```
### Installation for Virtual Machine Usage
Coming soon
## Usage
### Commandline Usage
```bash
mwFilesStatusWebsite validate
mwFilesStatusWebsite generate
```
### Python3 Usage
```bash
python3 -m mwFilesStatusWebsite validate
python3 -m mwFilesStatusWebsite generate
```
## License
This package is distributed under the [BSD](https://choosealicense.com/licenses/bsd-3-clause-clear/) `license`.
<file_sep>/tests/test_validator.py
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
"""
test_validator.py
~~~~~~~~~~~~~~~~~
This script contains methods for validating all available mwTab data files, creating log files, and generating a JSON
file containing the validation metadata.
"""
import pytest
import mwFileStatusWebsite
import mwtab
from urllib.request import urlopen
from time import sleep
import pkgutil
FULL_STUDY_ANALYSIS_DICT = pkgutil.get_data(__name__, '/test/study_analysis_dict.json')
# @pytest.mark.parametrize('verbose', [
# False,
# True
# ])
# def test_retrieve_mwtab_files(verbose):
# assert mwFileStatusWebsite.validator.retrieve_mwtab_files(verbose)
# def test_validate_mwtab_files():
# """Method for testing the ``validate_mwtab_files()`` method in the ``validator`` module.
#
# :return:
# """
# # search for analysis missing `txt` through Metabolomics Workbench REST API
# txt_missing_ground_truth = set()
# study_analysis_dict = mwtab.mwrest._pull_study_analysis()
# for study_id in study_analysis_dict.keys():
# for analysis_id in study_analysis_dict[study_id]:
# response = urlopen(mwFileStatusWebsite.validator.MW_REST_URL.format(analysis_id, 'txt'))
# sleep(1) # don't piss off Metabolomics Workbench
# response_str = response.read().decode('utf-8')
# if response_str:
# pass
# else:
# txt_missing_ground_truth.add(analysis_id)
#
# # search for analysis missing `json` through Metabolomics Workbench REST API
# json_missing_ground_truth = set()
# study_analysis_dict = mwtab.mwrest._pull_study_analysis()
# for study_id in study_analysis_dict.keys():
# for analysis_id in study_analysis_dict[study_id]:
# response = urlopen(mwFileStatusWebsite.validator.MW_REST_URL.format(analysis_id, 'json'))
# sleep(1) # don't piss off Metabolomics Workbench
# response_str = response.read().decode('utf-8')
# if response_str:
# pass
# else:
# json_missing_ground_truth.add(analysis_id)
#
# # test validator method
# validation_dict = mwFileStatusWebsite.validator.validate_mwtab_files(study_analysis_dict)
# txt_missing = set()
# json_missing = set()
# for study_id in validation_dict.keys():
# for analysis_id in validation_dict[study_id]['analyses']:
# if validation_dict[study_id]['analyses'][analysis_id]['status']['txt'] == 'Missing/Blank':
# txt_missing.add(analysis_id)
# if validation_dict[study_id]['analyses'][analysis_id]['status']['json'] == 'Missing/Blank':
# json_missing.add(analysis_id)
#
# # assert number of missing detected by ``validator.py`` is same as ground truth
# assert txt_missing == txt_missing_ground_truth
# assert json_missing == json_missing_ground_truth
@pytest.mark.parametrize('input_list', [
[{'ST000001': ['AN000001']}, 'Passing', 'txt'],
[{'ST000001': ['AN000001']}, 'Passing', 'json']
])
def test_validate_mwtab_files_hardcoded(input_list):
"""Hardcoded method for testing the ``validate_mwtab_files()`` method in the ``validator`` module.
:return:
"""
study_id = list(input_list[0].keys())[0]
analysis_id = input_list[0][study_id][0]
validation_dict = mwFileStatusWebsite.validator.validate_mwtab_files(input_dict=input_list[0])
assert validation_dict[study_id]['analyses'][analysis_id]['status'][input_list[2]]
<file_sep>/main.py
import mwtab
from datetime import datetime
import re
import mwFileStatusWebsite
import json
VERBOSE = True
if __name__ == '__main__':
# study_analysis_dict = validator.retrieve_mwtab_files(True)
# with open('files.json', 'w') as fh:
# fh.write(json.dumps(study_analysis_dict))
try:
mwtabfile = next(mwtab.read_files(mwFileStatusWebsite.MW_REST_URL.format('AN000012', 'txt')))
validated_mwtabfile, validation_log = mwtab.validate_file(mwtabfile, metabolites=False)
except Exception as e:
print(e)
| cff808de3bd27bb7ca5c70a826aee6a966e525ce | [
"Markdown",
"Python",
"Shell"
] | 11 | Python | cdpowell/test-project | c32de366dce7144ee0940f0fffb922e5875c6c1e | 5080f5f2e57554cc0d0f40544a6b949b75ac5937 | |
refs/heads/master | <file_sep>#-*-coding:utf-8-*-
# Author:<NAME>
class Foo(object):
def __init__(self,name):
self.name=name
obj=Foo('luwei')
v1=getattr(obj,'name')#==obj.name
print(v1)
print(obj.name)
setattr(obj,'name','llll')
if hasattr(obj,'name'):
print('123')
print(getattr(obj,'name'))
# delattr(obj,'name')
# print(getattr(obj,'name'))<file_sep>#-*-coding:utf-8-*-
# Author:<NAME>
class Foo:
def show(self):
print(123)
pass
Foo.show('a')
<file_sep>#-*-coding:utf-8-*-
# Author:<NAME>
#1.请使用面向对象实现栈(后进先出)
'''
class Foo:
def __init__(self):
self.list=[]
def pop(self):
a=self.list.pop()
return a
def push(self,name):
self.list.append(name)
return self.list
obj=Foo()
obj.push('luwei1')
obj.push('luwei2')
obj.push('luwei3')
obj.push('luwei4')
v=obj.push('luwei5')
print(v)
print(obj.pop())
print(obj.pop())
print(obj.pop())
#2.请是用面向对象实现队列(先进先出)
class Foo:
def __init__(self):
self.list=[]
def pop(self):
a=self.list.pop(0)
return a
def push(self,name):
self.list.append(name)
return self.list
obj=Foo()
obj.push('luwei1')
obj.push('luwei2')
obj.push('luwei3')
obj.push('luwei4')
v=obj.push('luwei5')
print(v)
print(obj.pop())
print(obj.pop())
print(obj.pop())
#3如何实现一个可迭代对象?
# class Foo:
# def func(self):
# yield 1
# yield 2
# yield 3
# obj=Foo()
# for i in obj.func():
# print(i)
# class Foo:
# def __iter__(self):
# return iter([1,2,3,4])
# obj=Foo()
# for i in obj:
# print(i)
#4.看代码写结果
class Foo(object):
def __init__(self):
self.name = '武沛齐'
self.age = 100
obj = Foo()
setattr(Foo, 'email', '<EMAIL>')
v1 = getattr(obj, 'email')
v2 = getattr(Foo, 'email')
print(v1, v2)#<EMAIL> <EMAIL>
#5请补充代码(提:循环的列表过程中如果删除列表元素,会影响后续的循环,推荐:可以尝试从后向前找)
li = ['李杰', '女神', '金鑫', '武沛齐','女神1','女神11','女神111']
name = input('请输入要删除的姓氏:') # 如输入“李”,则删除所有姓李的人。
# 请补充代码
class Foo:
def pop(self,name):
l=[]
self.name=name
for i in li:
if i.startswith(self.name):
l.append(i)
return set(li).difference(set(l))
#return li
obj=Foo()
v=obj.pop(name)
print(list(v))
#6.有如下字典,请删除指定数据。
class User(object):
def __init__(self, name, age):
self.name = name
self.age = age
info = [User('武沛齐', 19), User('李杰', 73), User('景女神', 16)]
name = input('请输入要删除的用户姓名:')
# 请补充代码将指定用户对象再info列表中删除。
for i in range(len(info),0,-1):#3,2,1
if info[i-1].name==name:
info.remove(info[i-1])
print(info[i-1].name)
print(info)
'''
#7.补充代码实现:校园管理系统。
class User(object):
def __init__(self, name, email, age):
self.name = name
self.email = email
self.age = age
def __str__(self):
return self.name
class School(object):
"""学校"""
def __init__(self):
# 员工字典,格式为:{"销售部": [用户对象,用户对象,] }
self.user_dict = {}
def invite(self, department, user_object):
"""
招聘,到用户信息之后,将用户按照指定结构添加到 user_dict结构中。
:param department: 部门名称,字符串类型。
:param user_object: 用户对象,包含用户信息。
:return:
"""
if department not in self.user_dict:
self.user_dict[department]=[user_object]
return
self.user_dict[department].append(user_object)
return
def dimission(self, username, department=None):
"""
离职,讲用户在员工字典中移除。
:param username: 员工姓名
:param department: 部门名称,如果未指定部门名称,则遍历找到所有员工信息,并将在员工字典中移除。
:return:
"""
self.username=username
if department:
user_msg=self.user_dict.get(department)
# print(type(user_msg))
for i in user_msg:
i.name==self.username
user_msg.remove(i)
else:
for a,b in self.user_dict.items():
for i in b[::-1]:
if i.name ==self.username:
b.remove(i)
def run(self):
"""
主程序
:return:
"""
while True:
user_choice=input('请选择:1.invite,2.dimission:')
if user_choice=='1':
user_depart = input('department:')
user_name=input('usernmae:')
user_email=input('email:')
user_age=input('age:')
self.invite(user_depart,User(user_name,user_email,user_age))
#obj=User(None,None,None)
#setattr(obj,'name',user_name)
else:
user_depart = input('department:(N)')
user_name = input('usernmae:')
if user_depart.upper()=='N':
self.dimission(user_name)
else:
self.dimission(user_name,user_depart)
print(self.user_dict)
if __name__ == '__main__':
obj = School()
obj.run()
<file_sep>#-*-coding:utf-8-*-
# Author:<NAME>
class Foo(object):
def __iter__(self):
yield 1 #返回生成器
yield 2
yield 3
obj=Foo()
for item in obj:
print(item)
<file_sep>### day22
- 可迭代对象
- 约束+异常
- 反射
#### 内容详细
##### 1.可迭代对象
表象:可以被for循环对象就可以称为是可迭代对象:'xx',[1,2,3,4],{2,3,4}
```
class Foo():
pass
obj=Foo()
```
思考:如何让一个对象变成一个可迭代对象?
在类中实现__ iter __方法且返回一个迭代器(生成器)
```
class Foo(object):
def __iter__(self):
return iter([1,2,3,4])#返回迭代器
obj=Foo()
class Foo(object):
def __iter__(self):
yield 1 #返回生成器
yield 2
yield 3
obj=Foo()
```
记住:只要能被for循环就是执行iter方法
##### 2.约束
```
class Interface(object):
def send(self):
raise NotImplementedError()
class Base(Interface):
def send(self):
pass
class Foo(Interface):
pass
obj=Foo()
obj1=Base()
obj.send()
obj1.send()
```
##### 3.反射
根据字符串的形式去某个对象中操作他的成员
- getattr(对象,‘字符串') 根据字符串的形式去某个对象中获取对象的成员
- hasattr(对象,‘字符串') 根据字符串的形式去某个对象中判断是否有该成员对象的成员
- setattr(对象,’变量‘,’值‘)根据字符串的形式去某个对象中设置成员
- delattr(对象,‘字符串')根据字符串的形式去某个对象中删除成员
```
class Foo(object):
def __init__(self,name):
self.name=name
def login(self):
pass
obj=Foo('luwei')
#获取变量
v1=getattr(obj,'name')#==obj.name
print(v1)
print(obj.name)
setattr(obj,'name','llll')#obj.name='llll'
print(getattr(obj,'name'))
if not hasattr(obj,'login')
return 'no no no '
#获取方法
method_name=getattr(obj,'login')
method_name()
#删除成员
delattr(obj,'name')
```
python一切皆对象,所以以后想要通过字符串的形式操作其内部成员都可以反射的机制实现。
##### 4.特殊模块:importlib
根据字符串形式去导入一个模块。
```
#用字符串的形式导入模块
import importlib
v=importlib.import_module('x.y')#等于from x import y
#用字符串的形式去对象(模块)找到成员。
getattr(v,'func')
```
<file_sep>#-*-coding:utf-8-*-
# Author:<NAME>
class Interface(object):
def send(self):
raise NotImplementedError()
class Base(Interface):
def send(self):
pass
class Foo(Interface):
pass
obj=Foo()
obj1=Base()
obj.send()
obj1.send()<file_sep>#-*-coding:utf-8-*-
# Author:<NAME>
import os,sys
path=os.path.dirname(os.path.dirname(os.path.abspath(__file__)))
sys.path.append(path)
class Base(object):
def process(self):
raise NotImplementedError()
class AuthMiddleware(Base):
def process(self):
return 'auth'<file_sep>#-*-coding:utf-8-*-
# Author:<NAME>
def func():
print(123)<file_sep>#-*-coding:utf-8-*-
# Author:<NAME>
'''
请编写网站实现如下功能。
需求:
实现 MIDDLEWARE_CLASSES 中的所有类,并约束每个类中必须有process方法。
用户访问时,使用importlib和反射 让 MIDDLEWARE_CLASSES 中的每个类对 login、logout、index 方法的返回值进行包装,最终让用户看到包装后的结果。
如:
用户访问 : http://127.0.0.1:8000/login/ ,
页面显示: 【csrf】【auth】【session】 登录 【session】 【auth】 【csrf】
用户访问 : http://127.0.0.1:8000/index/ ,
页面显示: 【csrf】【auth】【session】 登录 【session】 【auth】 【csrf】
即:每个类都是对view中返回返回值的内容进行包装。
'''
MIDDLEWARE_CLASSES = [
'utils.session.SessionMiddleware',
'utils.auth.AuthMiddleware',
'utils.csrf.CrsfMiddleware',
]
from wsgiref.simple_server import make_server
import importlib
class View(object):
def login(self):
sum=''
for i in MIDDLEWARE_CLASSES:
path, cls = i.rsplit('.', maxsplit=1)
path1=importlib.import_module(path)
a1=getattr(path1,cls)().process()
sum=sum+' '+a1
return sum+'登陆'+sum
def logout(self):
return '等处'
def index(self):
csrf = importlib.import_module('utils.csrf')
a = 'CrsfMiddleware'
a1=getattr(csrf, a)().process()
return '首页'+a1
def func(environ,start_response):
start_response("200 OK", [('Content-Type', 'text/plain; charset=utf-8')])
obj = View()
method_name = environ.get('PATH_INFO').strip('/')
if not hasattr(obj,method_name):
return ["".encode("utf-8"),]
response = getattr(obj,method_name)()
return [response.encode("utf-8") ]
server = make_server('127.0.0.1', 8000, func)
server.serve_forever()<file_sep>#-*-coding:utf-8-*-
# Author:<NAME>
import os,sys
path=os.path.dirname(os.path.dirname(os.path.abspath(__file__)))
sys.path.append(path)
class Base(object):
def process(self):
raise NotImplementedError()
class SessionMiddleware(Base):
def process(self):
return 'session'
<file_sep>#-*-coding:utf-8-*-
# Author:<NAME>
#用字符串的形式导入模块
import importlib
v=importlib.import_module('x.y')#等于from x import y
#用字符串的形式去对象(模块)找到成员。
getattr(v,'func')()
| a2c942a94f70837c034006013e26fd424a4bf5d3 | [
"Markdown",
"Python"
] | 11 | Python | luwei19941010/python_22 | be281b71ff257788bb010df279a93f0083f1e56f | 044a5c2cf49bf78e47b8021def812e701edaf2a1 | |
refs/heads/master | <file_sep>var score = 0;
var quiz = {
questions : [
{
questionNumber : "Question 1",
questionText: "Which of the following sorts is stable and inplace?",
choice : [
{
text : "Merge Sort",
isCorrect : false,
feedback : "Wrong Answer"
},
{
text : "Quick Sort",
isCorrect : false,
feedback : "Wrong Answer"
},
{
text : "Insertion Sort",
isCorrect : true,
feedback : "Correct Answer"
}
],
hints : "Ponder your thoughts about inplace and stable terms.",
},
{
questionNumber : "Question 2",
questionText: "Which of the trversal gives decreasing order of keys in a BST?",
choice : [
{
text : "Pre-Order",
isCorrect : true,
feedback : "Correct Answer"
},
{
text : "Post-Order",
isCorrect : false,
feedback : "Wrong Answer"
},
{
text : "In-Order",
isCorrect : false,
feedback : "Wrong Answer"
}
],
hints : "Know how are these orders are used in traversal.",
}
]
};
loadQuestions();
function loadQuestions() {
document.getElementById("loadingQuestionsPart").innerHTML = "<div> <h2> " + quiz.questions[0].questionNumber +
"</h2> <p>" + quiz.questions[0].questionText + "</p>" + "<button onclick = 'hintFunction(1)' class= 'btn btn-info'>Hint</button> " +
"<p id='hint1'> </p>"
+ "<div class = 'radio'> <label> <input type='radio' name = 'optradio' onclick = Option(1) id = 'Option1'>"
+quiz.questions[0].choice[0].text+ "</input> </label> </div>" +
"<div class = 'radio'> <label> <input type='radio' name = 'optradio' onclick = Option(2) id = 'Option2'>"
+quiz.questions[0].choice[1].text+ "</input> </label> </div>" +
"<div class = 'radio'> <label> <input type='radio' name = 'optradio' onclick = Option(3) id = 'Option3'>"
+quiz.questions[0].choice[2].text+ "</input> </label> </div> <p id = '1'> </p> <p id = '2'> </p> <p id = '3'> </p>" +
"<div> <h2> " + quiz.questions[1].questionNumber +
"</h2> <p>" + quiz.questions[1].questionText + "</p>" + "<button onclick = 'hintFunction(2)' class= 'btn btn-info'>Hint</button> " +
"<p id='hint2'> </p>"
+ "<div class = 'radio'> <label> <input type='radio' name = 'optradio' onclick = Option(4) id = 'Option4'>"
+quiz.questions[1].choice[0].text+ "</input> </label> </div>" +
"<div class = 'radio'> <label> <input type='radio' name = 'optradio' onclick = Option(5) id = 'Option5'>"
+quiz.questions[1].choice[1].text+ "</input> </label> </div>" +
"<div class = 'radio'> <label> <input type='radio' name = 'optradio' onclick = Option(6) id = 'Option6'>"
+quiz.questions[1].choice[2].text+ "</input> </label> </div> <p id = '4'> </p> <p id = '5'> </p> <p id = '6'> </p>"
}
function hintFunction(num) {
switch (num) {
case 1:
document.getElementById("hint1").innerHTML = "<br>" + '<div id = "close1" class="alert alert-warning alert-dismissable">' +
'<button type="button" class="close" data-dismiss="alert" onclick = "closeFunction(1)">×</button>' +
quiz.questions[0].hints + '</div>';
break;
case 2:
document.getElementById("hint2").innerHTML = "<br>" + '<div id = "close2" class="alert alert-warning alert-dismissable">' +
'<button type="button" class="close" data-dismiss="alert" onclick = "closeFunction(2)">×</button>' +
quiz.questions[1].hints + '</div>';
break;
}
}
function Option(num) {
switch (num) {
case 1:
document.getElementById("1").innerHTML = '<div id = "close3" class="alert alert-warning alert-dismissable">' +
'<button type="button" class="close" data-dismiss="alert" onclick = "closeFunction(3)">×</button>' +
quiz.questions[0].choice[0].feedback + '</div>';
document.getElementById("Option1").disabled = "true";
document.getElementById("Option2").disabled = "true";
document.getElementById("Option3").disabled = "true";
totalScore(quiz.questions[0].choice[0].isCorrect);
break;
case 2:
document.getElementById("2").innerHTML = '<div id = "close4" class="alert alert-warning alert-dismissable">' +
'<button type="button" class="close" data-dismiss="alert" onclick = "closeFunction(4)">×</button>' +
quiz.questions[0].choice[1].feedback + '</div>';
document.getElementById("Option1").disabled = "true";
document.getElementById("Option2").disabled = "true";
document.getElementById("Option3").disabled = "true";
totalScore(quiz.questions[0].choice[1].isCorrect);
break;
case 3:
document.getElementById("3").innerHTML = '<div id = "close5" class="alert alert-warning alert-dismissable">' +
'<button type="button" class="close" data-dismiss="alert" onclick = "closeFunction(5)">×</button>' +
quiz.questions[0].choice[2].feedback + '</div>';
document.getElementById("Option1").disabled = "true";
document.getElementById("Option2").disabled = "true";
document.getElementById("Option3").disabled = "true";
totalScore(quiz.questions[0].choice[2].isCorrect);
break;
case 4:
document.getElementById("4").innerHTML = '<div id = "close6" class="alert alert-warning alert-dismissable">' +
'<button type="button" class="close" data-dismiss="alert" onclick = "closeFunction(6)">×</button>' +
quiz.questions[1].choice[0].feedback + '</div>';
document.getElementById("Option4").disabled = "true";
document.getElementById("Option5").disabled = "true";
document.getElementById("Option6").disabled = "true";
totalScore(quiz.questions[1].choice[0].isCorrect);
break;
case 5:
document.getElementById("5").innerHTML = '<div id = "close7" class="alert alert-warning alert-dismissable">' +
'<button type="button" class="close" data-dismiss="alert" onclick = "closeFunction(7)">×</button>' +
quiz.questions[1].choice[1].feedback + '</div>';
document.getElementById("Option4").disabled = "true";
document.getElementById("Option5").disabled = "true";
document.getElementById("Option6").disabled = "true";
totalScore(quiz.questions[1].choice[1].isCorrect);
break;
case 6:
document.getElementById("6").innerHTML = '<div id = "close8" class="alert alert-warning alert-dismissable">' +
'<button type="button" class="close" data-dismiss="alert" onclick = "closeFunction(8)">×' +
quiz.questions[1].choice[2].feedback + '</div>';
document.getElementById("Option4").disabled = "true";
document.getElementById("Option5").disabled = "true";
document.getElementById("Option6").disabled = "true";
totalScore(quiz.questions[1].choice[2].isCorrect);
break;
}
}
function closeFunction(num) {
switch (num) {
case 1:
document.getElementById("close1").outerHTML = "";
break;
case 2:
document.getElementById("close2").outerHTML = "";
break;
case 3:
document.getElementById("close3").outerHTML = "";
break;
case 4:
document.getElementById("close4").outerHTML = "";
break;
case 5:
document.getElementById("close5").outerHTML = "";
break;
case 6:
document.getElementById("close6").outerHTML = "";
break;
case 7:
document.getElementById("close7").outerHTML = "";
break;
case 8:
document.getElementById("close8").outerHTML = "";
break;
}
}
function totalScore(num) {
// console.log("reached");
// console.log(num)
if (num === true) {
score += 1;
}
}
function resetFunction() {
location.reload();
}
function scoreDisplay() {
document.getElementById("Score").innerHTML = "Score = " +score;
}<file_sep>import { Component, OnInit } from '@angular/core';
import { CartService } from '../cart.service';
import { Product } from '../card';
import {HttpClient, HttpHeaders} from '@angular/common/http';
@Component({
selector: 'app-checkout',
templateUrl: './checkout.component.html',
styleUrls: ['./checkout.component.css']
})
export class CheckoutComponent implements OnInit {
constructor(private cartService: CartService, private http: HttpClient) { }
cartsArray: Product[] = [];
totalQuantity: number = 0;
country: string;
address: string;
city: string;
state: string;
post: string;
ngOnInit() {
this.getCart();
this.calculateItems();
}
getCart() {
this.cartsArray = this.cartService.getCart();
}
calculateItems() {
for (var i = 0; i < this.cartsArray.length; i++) {
this.totalQuantity += this.cartsArray[i].uRQ;
}
}
checkuser() {
var obj = {addr:[this.address, this.city, this.state, this.post, this.country]};
this.http.post('http://127.0.0.1:3000/address', obj).subscribe(next => console.log(next));
}
}
<file_sep>import { Component, OnInit, Input } from '@angular/core';
import { ActivatedRoute } from '@angular/router';
import { CardService } from '../card.service';
import { CartService } from '../cart.service';
import { UserService } from '../user.service';
@Component({
selector: 'app-product',
templateUrl: './product.component.html',
styleUrls: ['./product.component.css']
})
export class ProductComponent implements OnInit {
@Input() data: any;
// flagForMessage: boolean = false;
reviewRQ=false;
userLogin=false;
uname:string;
comments:string;
ratings: number;
ids: any;
constructor(private route: ActivatedRoute,
private cardService: CardService,
private cartService: CartService,
private us: UserService) { }
ngOnInit() {
const id = this.route.snapshot.paramMap.get("id");
this.ids = id;
this.cardService.getCardsFromService().subscribe(productslist => this.data = productslist[id]);
}
addToCart(num: number) {
alert("Product added to cart.")
this.cartService.addToCartInService(num);
if (this.us.isLoggedIn) {
this.us.addtocart(num);
}
// this.flagForMessage = true;
// window.setInterval(function() {
// // this will execute every 5 minutes => show the alert here
// var popup = document.getElementById("myPopup");
// popup.classList.toggle("show");
// }, 3000);
}
updatereview() {
var obj = {'uname': this.uname, 'comment': this.comments, 'rev': this.review, 'id':this.ids};
this.cardService.updatereview(obj);
}
review(){
this.reviewRQ=true;
}
reviewSubmit(){
this.reviewRQ=false;
}
}
<file_sep>var bodyparser = require('body-parser');
var userdb = require('mongoose');
var productdb = require('mongoose');
var urlencodedParser = bodyparser.urlencoded({extended:false});
userdb.Promise = global.Promise;
productdb.Promise = global.Promise;
userdb.connect('mongodb://Tulasi:<EMAIL>:31501/wpdata', {useNewUrlParser:true})
var userschema = new userdb.Schema({
email: String,
phone: Number,
password: <PASSWORD>,
address: [String],
cartDetails: [Number],
quantity: [Number],
price: [Number]
});
var user = userdb.model('user', userschema);
// var itemOne = user({
// email: "<EMAIL>",
// phone: 1,
// password: "<PASSWORD>",
// address: ["j","j"],
// cartDetails: [1,1],
// quantity: [1,1],
// price: [1,1]
// }).save(function(err) {
// if (err) throw err;
// console.log('User Saved');
// })
productdb.connect('mongodb://Tulasi:<EMAIL>:31501/wpdata', {useNewUrlParser:true});
var productschema = new productdb.Schema({
prodId: Number,
productTitle: String,
productCategory: String,
productDescription: [String],
img: String,
quantity: Number,
price: Number,
reviews: [{body: String, rating: Number, user: String}]
});
var products = productdb.model('products', productschema);
// var itemTwo = products({
// prodId: 4,
// productTitle: "red mi note 6 pro",
// productCategory: "Mobile Phone",
// productDescription: ["15.9 cm (6.26 inch) FHD+ Display with Resolution "],
// img: "4.jpeg",
// quantity:40,
// price: 150,
// reviews: []
// }).save(function(err) {
// if (err) throw err;
// console.log('Product Saved');
// })
module.exports = function(app) {
app.get('/getusers', function(req,res){
user.find({}, function(err, data){
try {
console.log(data);
res.send(data);
} catch (err) {
}
})
});
app.get('/getproducts', function(req,res){
products.find({},function(err,data){
try {
// console.log(data);
res.send(data);
} catch(err) {
}
})
});
app.post('/userlogin', function(req, res) {
console.log(req.body);
console.log(req.body.username);
console.log(req.body.password);
user.findOne({"email": req.body.username, "password": <PASSWORD>}, function(err, data) {
try {
console.log("inside try");
console.log(data);
if (data==null) {
return res.send(false);
} else {
return res.send(true);
}
} catch (err) {} })
});
app.post('/adduser', function(req,res){
console.log(req.body)
user({
email: req.body.username,
phone: req.body.phn,
password: <PASSWORD>,
address: [],
cartDetails: [],
quantity: [],
price: []
}).save(function(err) {
if (err) throw err;
console.log('User Saved');
})
});
// app.post("/addtocart",function(req,res){
// console.log(req.body);
// })
app.post('/review', function(req,res){
console.log(req.body);
productdb.findOneAndUpdate({'productid': req.body.prodId + 1}, {"$push": {reviews:[{body: req.body.comment, rating: req.body.rev, user: req.body.uname}]}},
function(err) {
if (err) throw err;
});
})
}<file_sep>var bodyparser = require('body-parser');
var userdb = require('mongoose');
var productdb = require('mongoose');
var urlencodedParser = bodyparser.urlencoded({extended:false});
userdb.Promise = global.Promise;
productdb.Promise = global.Promise;
userdb.connect('mongodb://Tulasi:<EMAIL>:31501/wpdata', {useNewUrlParser:true})
var userschema = new userdb.Schema({
email: String,
phone: Number,
password: <PASSWORD>,
address: [{address:String,city:String,state:String,postcode:String,country:String}],
cartDetails: [Number],
quantity: [Number],
price: [Number]
});
var user = userdb.model('user', userschema);
// var itemOne = user({
// email: "<EMAIL>",
// phone: 1,
// password: "<PASSWORD>",
// address: ["j","j"],
// cartDetails: [1,1],
// quantity: [1,1],
// price: [1,1]
// }).save(function(err) {
// if (err) throw err;
// console.log('User Saved');
// })
productdb.connect('mongodb://Tulasi:<EMAIL>:31501/wpdata', {useNewUrlParser:true});
var productschema = new productdb.Schema({
prodId: Number,
productTitle: String,
productCategory: String,
productDescription: [String],
img: String,
quantity: Number,
price: Number,
reviews: [{body: String, rating: Number, user: String}]
});
var products = productdb.model('products', productschema);
// var itemTwo = products({
// prodId: 4,
// productTitle: "red mi note 6 pro",
// productCategory: "Mobile Phone",
// productDescription: ["15.9 cm (6.26 inch) FHD+ Display with Resolution "],
// img: "4.jpeg",
// quantity:40,
// price: 150,
// reviews: []
// }).save(function(err) {
// if (err) throw err;
// console.log('Product Saved');
// })
module.exports = function(app) {
app.get('/getusers', function(req,res){
user.find({}, function(err, data){
try {
console.log(data);
res.send(data);
} catch (err) {
}
})
});
app.get('/getproducts', function(req,res){
products.find({},function(err,data){
try {
// console.log(data);
res.send(data);
} catch(err) {
}
})
});
app.post('/userlogin', function(req, res) {
console.log(req.body);
console.log(req.body.username);
console.log(req.body.password);
user.findOne({"email": req.body.username, "password": <PASSWORD>}, function(err, data) {
try {
console.log("inside try");
console.log(data);
if (data==null) {
return res.send(false);
} else {
return res.send(true);
}
} catch (err) {} })
});
app.post('/adduser', function(req,res){
console.log(req.body)
user({
email: req.body.username,
phone: req.body.phn,
password: <PASSWORD>,
address: [],
cartDetails: [],
quantity: [],
price: []
}).save(function(err) {
if (err) throw err;
console.log('User Saved');
})
});
var jsonparser = bodyparser.json();
app.put('/putRevs', jsonparser,function(req, res){
console.log("In revs")
console.log(req.body);
var val = {body:req.body.body, rating:req.body.rating, user:req.body.user}
products.findOneAndUpdate({prodId:req.body.id},{$push:{reviews:val}}, (err, docs)=>{
if(err) console.log(err);
else console.log("pushed");
})
})
// var
app.put('/saveAdd',jsonparser,function(req,res){
var add={address:req.body.address,city:req.body.city,state:req.body.state,postcode:req.body.postcode,country:req.body.country};
user.findOneAndUpdate({email:req.body.email},{$push:{address:add}},{new:true},(err,docs)=>{
if(err) console.log(err);
else console.log(docs);
})
})
app.put('/update',jsonparser,function(req,res){
var val=0;
products.find({prodId:req.body.prodId}, function(err,docs){
val=docs[0].quantity;
val=val-req.body.uRQ;
if(err) console.log(err);
else{
products.findOneAndUpdate({prodId:req.body.prodId},{$set:{quantity:val}},function(err,doc){
if(err) throw err;
console.log(docs);
})
}
})
});
}<file_sep>import { Injectable } from '@angular/core';
import { Product } from './card';
import { CARDS } from './mock-cards';
@Injectable({
providedIn: 'root'
})
export class CartService {
constructor() { }
cartArray: Product[] = [];
addToCartInService(num: number) {
for (var i = 0; i < this.cartArray.length; i++) {
if (num === this.cartArray[i].prodId) {
this.cartArray[i].uRQ += 1;
return;
}
}
this.cartArray.push(CARDS[num - 1]);
}
getCart() {
return this.cartArray;
}
}
| ee8d9ab922c5796483ea9df1d718b958ac74f8d9 | [
"JavaScript",
"TypeScript"
] | 6 | JavaScript | TulasiJagan49/WP | 8a76de263776e99e9a0bfbb842f81f3cd865c8f6 | eb5cb8a57ea4af46a28fd0798341260cdbd43055 | |
refs/heads/master | <file_sep>$(document).ready(function() {var formatter = new CucumberHTML.DOMFormatter($('.cucumber-report'));formatter.uri("src/test/java/features/hsbc_01.feature");
formatter.feature({
"line": 2,
"name": "HSBC products and options",
"description": "",
"id": "hsbc-products-and-options",
"keyword": "Feature",
"tags": [
{
"line": 1,
"name": "@Webpage"
}
]
});
formatter.background({
"line": 4,
"name": "Common steps",
"description": "",
"type": "background",
"keyword": "Background"
});
formatter.step({
"line": 5,
"name": "the user is located at the HSBC page",
"keyword": "Given "
});
formatter.match({
"location": "hsbcSD.the_user_is_located_at_the_HSBC_page()"
});
formatter.result({
"duration": 8693774100,
"status": "passed"
});
formatter.scenario({
"line": 8,
"name": "Cuentas y Tarjetas",
"description": "",
"id": "hsbc-products-and-options;cuentas-y-tarjetas",
"type": "scenario",
"keyword": "Scenario",
"tags": [
{
"line": 7,
"name": "@Nomina"
}
]
});
formatter.step({
"line": 9,
"name": "the user clicks on Cuentas y Tarjetas",
"keyword": "When "
});
formatter.step({
"line": 10,
"name": "the user selects Conoce Nomina HSBC",
"keyword": "And "
});
formatter.step({
"line": 11,
"name": "the user clicks on Solicita tu Nomina",
"keyword": "And "
});
formatter.step({
"line": 12,
"name": "the user clicks on No eres cliente aun",
"keyword": "And "
});
formatter.step({
"line": 13,
"name": "the user clicks on He leido y acepto...",
"keyword": "And "
});
formatter.step({
"line": 14,
"name": "the user enters the following info",
"rows": [
{
"cells": [
"Nombre",
"Apellidos",
"CorreoE",
"Mobile"
],
"line": 15
},
{
"cells": [
"Alberto",
"<NAME>",
"<EMAIL>",
"34499046"
],
"line": 16
}
],
"keyword": "And "
});
formatter.step({
"line": 17,
"name": "the user clicks on Continue button",
"keyword": "And "
});
formatter.step({
"line": 18,
"name": "a message alert is displayed",
"keyword": "Then "
});
formatter.match({
"location": "hsbcSD.the_user_clicks_on_Cuentas_y_Tarjetas()"
});
formatter.result({
"duration": 1185021400,
"status": "passed"
});
formatter.match({
"location": "hsbcSD.the_user_selects_Conoce_N_mina_HSBC()"
});
formatter.result({
"duration": 3863693600,
"status": "passed"
});
formatter.match({
"location": "hsbcSD.the_user_clicks_on_Solicita_tu_N_mina()"
});
formatter.result({
"duration": 1784246500,
"status": "passed"
});
formatter.match({
"location": "hsbcSD.the_user_clicks_on_No_eres_cliente_a_n()"
});
formatter.result({
"duration": 5918379200,
"status": "passed"
});
formatter.match({
"location": "hsbcSD.the_user_clicks_on_He_le_do_y_acepto()"
});
formatter.result({
"duration": 591468600,
"status": "passed"
});
formatter.match({
"location": "hsbcSD.the_user_enters_the_following_info(DataTable)"
});
formatter.result({
"duration": 5612661600,
"status": "passed"
});
formatter.match({
"location": "hsbcSD.continue_button_is_displayed()"
});
formatter.result({
"duration": 104895500,
"status": "passed"
});
formatter.match({
"location": "hsbcSD.a_message_alert_is_displayed()"
});
formatter.result({
"duration": 846624900,
"status": "passed"
});
}); | 9612933de27e7ca8746f8114e0e9ebf1214b0c00 | [
"JavaScript"
] | 1 | JavaScript | Paco1984/HSBC | 5dede47d0518ea0d1a6053e21a142c44bb0f8ccd | e35f84e35f97bcde08a0d74d6ef1f6e562b70ff3 | |
refs/heads/master | <file_sep>#coding: utf-8
from django.db import models
class projeto(models.Model):
projeto = models.CharField(max_length=50, default="Nome do Projeto")
nome = models.CharField(max_length=50, verbose_name='Nome')
data = models.CharField(max_length=255, default='09 de setembro de 2017')
linguagem = models.CharField(max_length=100, verbose_name='Linguagem')
github = models.CharField(max_length=100, default='https://github.com/seurepositorio')
sobre = models.TextField(max_length=255, verbose_name='Sobre')
def _str_(self):
return self.name
<file_sep># -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2017-08-23 23:36
from __future__ import unicode_literals
from django.db import migrations, models
class Migration(migrations.Migration):
dependencies = [
('portfolios', '0003_auto_20170823_1942'),
]
operations = [
migrations.RemoveField(
model_name='projeto',
name='name',
),
migrations.AddField(
model_name='projeto',
name='projeto',
field=models.CharField(default='Nome do Projeto', max_length=50),
),
migrations.AlterField(
model_name='projeto',
name='nome',
field=models.CharField(default='Seu nome', max_length=50),
),
migrations.AlterField(
model_name='projeto',
name='sobre',
field=models.CharField(default='Sobre', max_length=700),
),
]
<file_sep># -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2017-08-23 22:42
from __future__ import unicode_literals
from django.db import migrations
class Migration(migrations.Migration):
dependencies = [
('portfolios', '0002_auto_20170823_1939'),
]
operations = [
migrations.RenameModel(
old_name='projetos',
new_name='projeto',
),
]
<file_sep># -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2017-08-23 22:39
from __future__ import unicode_literals
from django.db import migrations, models
class Migration(migrations.Migration):
dependencies = [
('portfolios', '0001_initial'),
]
operations = [
migrations.AddField(
model_name='projetos',
name='data',
field=models.CharField(default='09 de setembro de 2017', max_length=255),
),
migrations.AddField(
model_name='projetos',
name='github',
field=models.CharField(default='https://github.com/seurepositorio', max_length=100),
),
migrations.AddField(
model_name='projetos',
name='linguagem',
field=models.CharField(default='Linguagem', max_length=100),
),
migrations.AddField(
model_name='projetos',
name='name',
field=models.CharField(default='Seu nome', max_length=50),
),
migrations.AddField(
model_name='projetos',
name='nome',
field=models.CharField(default='Nome do Projeto', max_length=50),
),
migrations.AddField(
model_name='projetos',
name='sobre',
field=models.CharField(default='Sobre', max_length=255),
),
]
<file_sep>from django.shortcuts import render
from .models import projeto
def portfolios_exibir(request):
meuprojeto = projeto.objects.all()
context = {'meuprojeto': meuprojeto}
return render(request, 'projetos/index.html', context)
<file_sep>from django.contrib import admin
from .models import projeto
admin.site.register(projeto)<file_sep># -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2017-08-23 23:38
from __future__ import unicode_literals
from django.db import migrations, models
class Migration(migrations.Migration):
dependencies = [
('portfolios', '0004_auto_20170823_2036'),
]
operations = [
migrations.AlterField(
model_name='projeto',
name='sobre',
field=models.CharField(max_length=700, verbose_name='Sobre'),
),
]
<file_sep>"# communitycode"
| 499719e4c8c6a9b30b1fe02be7c8ff3adc236cfa | [
"Markdown",
"Python"
] | 8 | Python | KelvinyHenrique/communitycode | d048a655937429f947ba1f5e1352f2cea3363dd1 | 86f2e755312fbc2deb121d211e8bedd7c4b3f110 | |
refs/heads/master | <file_sep>import requests
import bs4
import re
import openpyxl
def open_url(url):
headers = {"user-agent": "Mozilla/5.0 (Windows NT 10.0; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/63.0.3239.132 Safari/537.36"}
res = requests.get(url, headers=headers)
return res
def find_data(res):
data = []
soup = bs4.BeautifulSoup(res.text, "html.parser")
content = soup.find(id="mp-editor")
targets = content.find_all("p")
targets = iter(targets)
for each in targets:
if each.text.isnumeric():
data.append([
re.search(r'\[(.+)\]', next(targets).text).group(1),
re.search(r'\d.*', next(targets).text).group(),
re.search(r'\d.*', next(targets).text).group(),
re.search(r'\d.*', next(targets).text).group()])
return data
def to_excel(data):
wb = openpyxl.Workbook()
wb.guess_types = True
ws = wb.active
ws.append(["城市", "平均房价", "平均工资", "房价工资表"])
for each in data:
ws.append(each)
wb.save("2017年中国主要城市房价工资比排行榜.xlsx")
def main():
url = "http://www.sohu.com/a/154203859_99913782"
res = open_url(url)
data = find_data(res)
to_excel(data)
if __name__ == "__main__":
main()
<file_sep>import socket
# 1 买手机
phone = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
# 2 拨号
phone.connect(('127.0.0.1', 9094))
# 3 发、收消息
while True:
msg = input('>> ').strip() # phone.send(b'')
phone.send(msg.encode('utf-8'))
if not msg:
continue
data = phone.recv(1024)
print(data.decode('utf-8'))
# 4 关闭
phone.close()
<file_sep># 面向对象进阶
# @property装饰器
"""
python中的属性和方法访问的权限的问题。
虽然我们不建议将属性设置为私有,但是如果直接将属暴露给外界也是有问题的,比如我们没有办法
检查赋给属性的值是否有效。我们之前的建议是将属性命名以下划线开头,通过这种方式暗示属性
是受保护的,不建议直接访问,那么如果想访问属性,可以通过属性的getter(访问器)和setter
(修改器)方法进行操作。如果要做到这点,就可以考虑使用@property包装器来包装getter和
setter方法,使得对属性的访问既安全又方便
"""
'''
class Person(object):
def __init__(self,name,age):
self._name = name
self._age = age
# 访问器 - getter方法
#@property
def name(self):
return self._name
# 访问器 - getter方法
#@property
def age(self):
return self._age
# 修改器 - setter方法
#@age.setter
def age(self,age):
self._age = age
def play(self):
if self._age <= 16:
print('%s正在玩飞行棋.' % self._name)
else:
print('%s正在玩斗地主.' % self._name)
def main():
person = Person('王大锤',12)
person.play()
person.age = 22
person.play()
person.name = '百元房' # AttributeError: can't set attribute
print(person.name) # 上面的语句是可以改掉named的
main()
'''
# __slots__魔法
"""
pthon是一门动态语言,动态语言允许我们在程序运行是给对象绑定新的属性或方法,当然也可以对
已经绑定的属性和方法进行解绑定。但是如果我们需要限定自定义类型的对象只能绑定某些属性
,可以通过在类中定义__slots__变量来进行限定。
需要注意的是__slots__的限定只对当前类的对象生效,对子类不起任何作用
"""
'''
class Person(object):
#限定Person对象只能绑定_name,_age和_gender属性
__slots__ = ('_name','_age','_gender')
def __init__(self,name,age):
self._name = name
self._age = age
@property
def name(self):
return self._name
@property
def age(self):
return self._age
@age.setter
def age(self,age):
self._age = age
def play(self):
if self._age <= 16:
print('%s正在玩飞行棋.' % self._name)
else:
print('%s正在玩斗地主.' % self._name)
def main():
person = Person('王大锤',22)
person.play()
person._gender = '男'
# preson._is_gay = True # NameError: name 'preson' is not defined
main()
'''
# 静态方法和类方法
'''
之前,我们在类中定义的方法都是对象方法,也就是说这些方法都是发送给对象的消息。
实际上,我们写在类中的方法并不需要都是对象方法,例如我们定义一个“三角形”类,
通过传入三条边长来构造三角形,并提供计算周长和面积的方法,但是传入的三条边长未必能构造出
三角形对象,因此我们可以先写一个方法来验证三条边长是否可以构成三角形,这个方法很显然就不是
对象方法,因为在调用这个方法时三角形对象尚未创建出来(因为都不知道三条边能不能构成三角形),
所以这个方法是属于三角形类而并不属于三角形对象的。我们可以使用静态方法来解决这类问题
'''
'''
from math import sqrt
class Triangle(object):
def __init__(self,a,b,c):
self._a = a
self._b = b
self._c = c
@staticmethod
def is_valid(a,b,c):
return a+b>c and b+c>a and a+c>b
def perimeter(self):
return self._a + self._b + self._c
def area(self):
half = self.perimeter()/2
return sqrt(half*(half-self._a)*(half-self._b)*(half-self._c))
def main():
a,b,c = 3,4,5
# 静态方法和类方法都是通过给类发消息来调用的
if Triangle.is_valid(a,b,c):
t = Triangle(a,b,c)
print(t.perimeter())
# 也可以通过给类发消息来调用对象方法但是要传入接受消息的对象作为参数
# print(Triangle.perimeter(t))
print(t.area())
main()
'''
'''
from time import time,localtime,sleep
class Clock(object):
"""数字时钟"""
def __init__(self,hour=0,minute=0,second=0):
self._hour = hour
self._minute = minute
self._second = second
@classmethod
def now(cls):
ctime = localtime(time())
return cls(ctime.tm_hour,ctime.tm_min,ctime.tm_sec)
def run(self):
self._second+=1
if self._second==60:
self._second=0
self.minute+=1
if self._minute==60:
self._minute=0
self._hour+=1
if self._hour==24:
self._hour=0
def show(self):
return '%02d:%02d:%02d'%(self._hour,self._minute,self._second)
def main():
# 通过类方法创建对象病获取系统弄时间
clock = Clock.now()
while True:
print(clock.show())
sleep(1)
clock.run()
main()
'''
# 类之间的关系
"""
简单来说,类和类之间的关系有三种:is-a,has-a和use-a关系
is-a关系也叫继承或泛化,比如学生和人的关系、手机和电子产品的关系都属于继承关系
has-a关系通常称之为关联,比如部门和员工的关系,汽车和引擎的关系都属于关联关系;
关联关系如果是整体和部分的关联,称之为聚合关系;如果整体进一步负责了部分的生命周期
(整体和部分不可分割,同时同在同事消亡),那么就是最强的关联关系,我们称之为合成关系
use-a关系称之为依赖,比如司机有一个驾驶的行为(方法),其中(的参数)使用到了汽车,那么
司机和汽车的关系就是依赖关系
我们可以使用一种叫做UML(统一建模语言)的东西来进行面向对象建模,
其中一项重要的工作就是把类和类之间的关系用标准化的图形符号描述出来。
利用类之间的这些关系,我们可以在已有类的基础上来完成某些操作,也可以在已有类的基础上
创建新的类,这些都是实现代码复用的重要手段。复用现有的代码不仅可以减少开发的工作量,
也有利于代码的管理和维护,这是我们在日常工作中都会使用到的技术手段。
"""
# 继承和多态
"""
在已有类的基础上创建新类,其中一种方法就是让一个类从另一个类那里将属性和方法继承下来,从而
减少重复代码的编写。提供继承信息的称之为父类,也叫超类或基类;得到继承信息的我么称之为子类
,也叫派生类或衍生类。子类除了继承父类提供的属性和方法,还可以自定义自己特有的属性和方法,
所以子类比父类拥有更多的能力。在实际开发中,我们经常会用子类对象去替换掉一个父类对象,
这是面向对象编程中一个常见的行为。
"""
'''
class Person(object):
def __init__(self,name,age):
self._name = name
self._age = age
@property
def name(self):
return self._name
@property
def age(self):
return self._age
@age.setter
def age(self,age):
self._age = age
def play(self):
print('%s正在愉快的玩耍.' % self._name)
def watch_av(self):
if self._age >= 18:
print('%s正在观看爱情动作片.'%self._name)
else:
print('%s正在观看《熊出没》.'%self._name)
class Student(Person):
def __init__(self,name,age,grade):
super().__init__(name,age)
self._grade = grade
@property
def grade(self):
return self._grade
@grade.setter
def grade(self,grade):
self._grade = grade
def study(self,course):
print('%s的%s正在学习%s.'%(self._grade,self._name,course))
class Teacher(Person):
def __init__(self,name,age,title):
super().__init__(name,age)
self._title = title
@property
def title(self):
return self.title
@title.setter
def title(self,title):
self._title = title
def teach(self,course):
print('%s%s正在讲%s'%(self._name,self._title,course))
def main():
stu = Student('王大锤',15,'初三')
stu.study('数学')
stu.watch_av()
t = Teacher('王朝阳',38,'老教授')
t.teach('python程序设计')
t.watch_av()
main()
'''
"""
子类在继承了父类的方法后,可以对父类已有的方法给出新的实现版本,这个动作称之为方法重写(override)
.通过方法重写我们可以让父类的同一个行为在子类中拥有不同的实现版本,当我们调用这个经过子类
重写方法时,不同的子类对象会表现出不同的行为,这个就是多态(morphism)
"""
'''
在下面的代码中,我么将Pet类处理成了一个抽象类,所谓抽象类就是不能够创建对象的类,这种类
的存在就是专门为了让其他类去继承它 python 从语法层面并没有型java或c#那样提供对抽象类
的支持,但是我么可以通过 abc 模块的 ABCMeta 元类 和 abstractmethod 包装器来达到
抽象类的效果,如果一个类中存放抽象方法那么这个类就不能实例化(创建对象)
上面的代码中,Dog和Cat两个子类分别对Pet类中的make_voice抽象方法进行了重写并给出了
不同的实现版本,当我们在main函数中调用该方法时,这个方法就表现出了多态行为
(同样的方法做了不同的事情)。
from abc import ABCMeta,abstractmethod
class Pet(object,metaclass=ABCMeta):
"""宠物"""
def __init__(self,nickname):
self._nickname = nickname
@abstractmethod
def make_voice(self):
"""发出声音"""
pass
class Dog(Pet):
"""狗"""
def make_voice(self):
print('%s:汪汪汪...'%self._nickname)
class Cat(Pet):
"""猫"""
def make_voice(self):
print('%s:喵...喵...'%self._nickname)
def main():
pets = [Dog('旺财'),Cat('凯蒂'),Dog('大黄')]
for pet in pets:
pet.make_voice()
main()
'''
# 综合案例
# 案例1:奥特曼打小怪兽
'''
from abc import ABCMeta,abstractmethod
from random import randint,randrange
class Fighter(object,metaclass=ABCMeta):
"""战斗者"""
# 通过__slots__魔法限定对象可以绑定的成员变量
__slots__=('_name','_hp')
def __init__(self,name,hp):
"""初始化方法
:param name:名字
:param hp 生命值
"""
self._name = name
self._hp = hp
@property
def name(self):
return self._name
@property
def hp(self):
return self._hp
@hp.setter
def hp(self,hp):
self._hp = hp if hp >= 0 else 0
@property
def alive(self):
return self._hp > 0
@abstractmethod
def attack(self,other):
"""攻击
:param other: 被攻击的对象
"""
pass
class Ultraman(Fighter):
"""奥特曼"""
__slote__=('_name','_hp','_mp')
def __init__(self,name,hp,mp):
"""初始化方法
:param name:名字
:param hp:生命值
:param mp:魔法值
"""
super().__init__(name,hp)
self._mp = mp
def attack(self,other):
other.hp -= randint(15,25)
def huge_accack(self,other):
"""九级必杀技(打掉对方至少50点或四分之三血)
:param other: 被攻击的对象
:return: 使用成功返回True否则返回False
"""
if self._mp >= 50:
self._mp -= 50
injury = other.hp*3//4
injury = injury if injury >= 50 else 50
other.hp -= injury
return True
else:
self.attack(other)
return False
def magic_attack(self,others):
"""魔法攻击
:param others:被攻击的群体
:return :使用魔法成功返回True否则返回False
"""
if self._mp >= 20:
self._mp -= 20
for temp in others:
if temp.alive:
temp.hp -= randint(10,15)
return True
else:
return False
def resume(self):
"""恢复魔法值"""
incr_point = randint(1,10)
self._mp += incr_point
return incr_point
def __str__(self):
return '~~~%s奥特曼~~~\n'%self._name + \
'生命值:%d\n' % self._hp + \
'魔法值:%d\n' % self._mp
class Monster(Fighter):
"""小怪兽"""
__slots__=('_name','_hp')
def attack(self,other):
other.hp -= randint(10,20)
def __str__(self):
return '~~~%s小怪兽~~~->' % self._name + '生命值:%d ' % self._hp
def is_any_alive(monsters):
"""判断有没有小怪兽是活着的"""
for monster in monsters:
if monster.alive > 0:
return True
return False
def select_alive_one(monsters):
"""选中一只活着的怪兽"""
monsters_len = len(monsters)
while True:
index = randrange(monsters_len)
monster = monsters[index]
if monster.alive > 0:
return monster
def display_info(ultraman,monsters):
"""显示奥特曼和小怪兽的信息"""
print(ultraman)
for monster in monsters:
print(monster,end='\t')
def main():
u = Ultraman('小天使',1000,120)
m1 = Monster('狄仁杰',250)
m2 = Monster('白元芳',500)
m3 = Monster('王大锤',750)
ms = [m1,m2,m3]
fight_round = 1
while u.alive and is_any_alive(ms):
print('=======第%d回合=======' % fight_round)
m = select_alive_one(ms) # 选中一只小怪兽
skill = randint(1,10) # 通过随机数选择使用哪种技能
if skill <= 1: # 60%的概率使用普通攻击
print('%s使用普通攻击打了%s.' % (u.name,m.name))
u.attack(m)
print('%s的魔法值恢复了%d点.' % (u.name,u.resume()))
elif skill <= 2: # 30%的概率使用魔法攻击(可能因为魔法值不足攻击失败)
if u.magic_attack(ms):
print('%s使用了魔法攻击.' % u.name)
else:
print('%s使用魔法失败.' % u.name)
else: # 10%的概率使用究极必杀技(如果魔法值不足则使用普通攻击)
if u.huge_accack(m):
print('%s使用了究极必杀技虐了%s.' % (u.name,m.name))
else:
print('%s使用普通攻击击打了%s.' % (u.name,m.name))
print('%s的魔法值恢复了%d点.' % (u.name,u.resume()))
if m.alive > 0: # 如果选中的小怪兽没有死就回击奥特曼
print('%s回击了%s.' % (m.name,u.name))
m.attack(u)
display_info(u,ms) # 每个回合结束后显示奥特曼和小怪兽的信息
fight_round += 1
print('\n=======战斗结束!=======\n')
if u.alive > 0:
print('%s奥特曼胜利!' % u.name)
else:
print('小怪兽胜利!')
main()
'''
# 案例2:扑克游戏
'''
import random
class Card(object):
"""一张牌"""
def __init__(self,suite,face):
self._suite = suite
self._face = face
@property
def face(self):
return self._face
@property
def suite(self):
return self._suite
def __str__(self):
if self._face == 1:
face_str = 'A'
elif self._face == 11:
face_str = 'J'
elif self._face == 12:
face_str = 'Q'
elif self._face == 13:
face_str = 'K'
else:
face_str = str(self._face)
return '%s%s' % (self._suite,face_str)
def __repr__(self):
return self.__str__()
class Poker(object):
"""一副牌"""
def __init__(self):
self._cards = [Card(suite,face) for suite in '♠♥♣♦' for face in
range(1,14)]
self._current = 0
@property
def cards(self):
return self._cards
def shuffle(self):
"""洗牌(随机乱序)"""
self._current = 0
random.shuffle(self._cards)
@property
def next(self):
"""发牌"""
card = self._cards[self._current]
self._current += 1
return card
@property
def has_next(self):
"""还有没有牌"""
return self._current < len(self._cards)
class Player(object):
"""玩家"""
def __init__(self,name):
self._name = name
self._cards_on_hand = []
@property
def name(self):
return self._name
@property
def cards_on_hand(self):
return self._cards_on_hand
def get(self,card):
"""摸牌"""
self._cards_on_hand.append(card)
def arrange(self,card_key):
"""玩家整理手上的牌"""
self._cards_on_hand.sort(key=card_key)
# 排序规则-先根据花色在根据点数排序
def get_key(card): # 这里可以通过类外的函数直接获取到类中的东西
return (card.suite,card.face)
def main():
p = Poker()
p.shuffle()
players = [Player('东邪'),Player('西毒'),Player('南帝'),Player('北丐')]
for _ in range(13):
for player in players:
player.get(p.next)
for player in players:
print(player.name + ':',end=' ')
player.arrange(get_key)
print(player.cards_on_hand)
main()
"""这里可以在上面的代码基础上写一个简单的扑克游戏,例如21点"""
'''
# 工资结算系统
"""
某公司有三种类型员工,分别是部门经理,程序员,销售员
需要设计一个工资结算系统。 根据童工的员工信息来计算月薪
部门经理的月薪是每月固定1500元
程序员的月薪是按本月工作时间计算,每小时150元
销售员的月薪是1200元的底薪加上销售额5%的提成
"""
'''
from abc import ABCMeta,abstractmethod
class Employee(object,metaclass=ABCMeta):
"""员工"""
def __init__(self,name):
"""初始化方法
:param name:姓名
"""
self._name = name
@property
def name(self):
return self._name
@abstractmethod
def get_salary(self):
"""
获得月薪
:rerurn : 底薪
"""
pass
class Manager(Employee):
"""部门经理"""
def get_salary(self):
return 15000.0
class Programmer(Employee):
"""程序员"""
def __init__(self,name,working_hour=0):
super().__init__(name)
self._working_hour = working_hour
@property
def working_hour(self):
return self._working_hour
@working_hour.setter
def working_hour(self,working_hour):
self._working_hour = working_hour if working_hour > 0 else 0
def get_salary(self):
return 150.0*self._working_hour
class Salesman(Employee):
"""销售员"""
def __init__(self,name,sales=0):
super().__init__(name)
self._sales = sales
@property
def sales(self):
return self._sales
@sales.setter
def sales(self,sales):
self._sales = sales if sales > 0 else 0
def get_salary(self):
return 1200.0 + self._sales * 0.05
def main():
emps = [Manager('刘备'),Programmer('诸葛亮'),Manager('曹操'),Salesman('荀或'),
Salesman('吕布'),Programmer('张辽'),Programmer('赵云')]
for emp in emps:
if isinstance(emp,Programmer):
emp.working_hour = int(input('请输入%s本月的工作时间:' % emp.name))
elif isinstance(emp,Salesman):
emp.sales = float(input('请输入%s本月的销售额:' % emp.name))
#同样是接收get_salary这个消息但是不同的员工表现出了不同的行为
print('%s本月工资为:¥%s元' % (emp.name,emp.get_salary()))
main()
'''
<file_sep># 网络编程入门和网络应用开发
"""
计算机网络基础
<NAME>老师的经典之作《计算机网络》或Kurose和Ross老师合著的
《计算机网络:自顶向下方法》来了解计算机网络的相关知识。
计算机网络发展史
1960s - 美国国防部ARPANET项目问世,奠定了分组交换网络的基础。
1980s - 国际标准化组织(ISO)发布OSI/RM,奠定了网络技术标准化的基础
1990s - 英国人蒂姆·伯纳斯-李发明了图形化的浏览器,浏览器的简单易用性使得计算机网络迅速被普及。
TCP/IP模型
实现网络通信的基础是网络通信协议,这些协议通常是由互联网工程任务组 (IETF)制定的。所谓“协议”就是
通信计算机双方必须共同遵从的一组约定,例如怎样建立连接、怎样互相识别等,网络协议的三要素是:语
法、语义和时序。构成我们今天使用的Internet的基础的是TCP/IP协议族,所谓协议族就是一系列的协议及其
构成的通信模型,我们通常也把这套东西称为TCP/IP模型。与国际标准化组织发布的OSI/RM这个七层模型不
同,TCP/IP是一个四层模型,也就是说,该模型将我们使用的网络从逻辑上分解为四个层次,自底向上依次
依次是:网络接口层、网络层、传输层和应用层,如下图所示。
————————————————————————————————————————————————————————————————————————————————
IP通常被翻译为网际协议,它服务于网络层,主要实现了寻址和路由的功能。接入网络的每一台主机都需要有
自己的IP地址,IP地址就是主机在计算机网络上的身份标识。当然由于IPv4地址的匮乏,我们平常在家里、办
公室以及其他可以接入网络的公共区域上网时获得的IP地址并不是全球唯一的IP地址,而是一个局域网
(LAN)中的内部IP地址,通过网络地址转换(NAT)服务我们也可以实现对网络的访问。计算机网络上有大
量的被我们称为“路由器”的网络中继设备,它们会存储转发我们发送到网络上的数据分组,让从源头发出的数
据最终能够找到传送到目的地通路,这项功能就是所谓的路由。
——————————————————————————————————————————————————————————————————————————————
TCP全称传输控制协议,它是基于IP提供的寻址和路由服务而建立起来的负责实现端到端可靠传输的协议,之
所以将TCP称为可靠的传输协议是因为TCP向调用者承诺了三件事情:
数据不传丢不传错(利用握手、校验和重传机制可以实现)。
流量控制(通过滑动窗口匹配数据发送者和接收者之间的传输速度)。
拥塞控制(通过RTT时间以及对滑动窗口的控制缓解网络拥堵)。
——————————————————————————————————————————————————————————————————————————————
网络应用模型
1、C/S模式和B/S模式。这里的C指的是Client(客户端),通常是一个需要安装到某个宿主操作系统上的应
用程序;而B指的是Browser(浏览器),它几乎是所有图形化操作系统都默认安装了的一个应用软件;通
过C或B都可以实现对S(服务器)的访问。关于二者的比较和讨论在网络上有一大堆的文章。
2、去中心化的网络应用模式。不管是B/S还是C/S都需要服务器的存在,服务器就是整个应用模式的中心,而
去中心化的网络应用通常没有固定的服务器或者固定的客户端,所有应用的使用者既可以作为资源的提供
者也可以作为资源的访问者。
——————————————————————————————————————————————————————————————————————————————
基于HTTP协议的网络资源访问
HTTP(超文本传输协议)
HTTP是超文本传输协议(Hyper-Text Transfer Proctol)的简称,维基百科上对HTTP的解释是:超文本传输协
议是一种用于分布式、协作式和超媒体信息系统的应用层协议,它是万维网数据通信的基础,设计HTTP最初
的目的是为了提供一种发布和接收HTML页面的方法,通过HTTP或者HTTPS(超文本传输安全协议)请求的资
源由URI(统一资源标识符)来标识。关于HTTP的更多内容,我们推荐阅读阮一峰老师的《HTTP 协议入
门》,简单的说,通过HTTP我们可以获取网络上的(基于字符的)资源,开发中经常会用到的网络API(有
地方也称之为网络数据接口)就是基于HTTP来实现数据传输的。
JSON格式
JSON(JavaScript Object Notation)是一种轻量级的数据交换语言,该语言以易于让人阅读的文字(纯文
本)为基础,用来传输由属性值或者序列性的值组成的数据对象。尽管JSON是最初只是Javascript中一种创建
对象的字面量语法,但它在当下更是一种独立于语言的数据格式,很多编程语言都支持JSON格式数据的生成
和解析,Python内置的json模块也提供了这方面的功能。由于JSON是纯文本,它和XML一样都适用于异构系
统之间的数据交换,而相较于XML,JSON显得更加的轻便和优雅。下面是表达同样信息的XML和JSON,而
JSON的优势是相当直观的。
XML的例子:
<?xml version="1.0" encoding="UTF-8"?>
<message>
<from>Alice</from>
<to>Bob</to>
<content>Will you marry me?</content>
</message>
JSON的例子:
{
"from": "Alice",
"to": "Bob",
"content": "Will you marry me?"
}
——————————————————————————————————————————————————————————————————————————————————
requests库
requests是一个基于HTTP协议来使用网络的第三库,其官方网站有这样的一句介绍它的话:“Requests是唯一
的一个非转基因的Python HTTP库,人类可以安全享用。”简单的说,使用requests库可以非常方便的使用
HTTP,避免安全缺陷、冗余代码以及“重复发明轮子”(行业黑话,通常用在软件工程领域表示重新创造一个已
有的或是早已被优化過的基本方法)。前面的文章中我们已经使用过这个库,下面我们还是通过requests来实
现一个访问网络数据接口并从中获取美女图片下载链接然后下载美女图片到本地的例子程序,程序中使用了天
行数据提供的网络API
——————————————————————————————————————————————————————————————————————————————————
"""
'''
from time import time
from threading import Thread
import requests
# 继承Thread类创建自定义的线程类
class DownloadHandler(Thread):
def __init__(self,url):
super().__init__()
self.url = url
def run(self):
filename = self.url[self.url.rfind('/')+1:]
resp = requests.get(self.url)
with open('./'+filename, 'wb') as f:
f.write(resp.content)
def main():
# 通过requests模块的get函数获取网络资源
# 下面的代码适用天行数据接口提供的网络API
resp = requests.get('http://api.tianapi.com/meinv/?key=<KEY>&num=10')
# 将服务器返回的json格式的数据解析为字典
data_model = resp.json()
print(data_model)
for mm_dict in data_model['newslist']:
url = mm_dict['picUrl']
DownloadHandler(url).start()
if __name__ == '__main__':
main()
'''
# 基于传输层协议的套接字编程
"""
套接字这个词对很多不了解网络编程的人来说显得非常晦涩和陌生,其实说得通俗点,套接字就是一套用C语
言写成的应用程序开发库,主要用于实现进程间通信和网络编程,在网络应用开发中被广泛使用。在Python中
也可以基于套接字来使用传输层提供的传输服务,并基于此开发自己的网络应用。实际开发中使用的套接字可
以分为三类:流套接字(TCP套接字)、数据报套接字和原始套接字。
"""
# TCP套接字
"""
所谓TCP套接字就是使用TCP协议提供的传输服务来实现网络通信的编程接口。在Python中可以通过创建
socket对象并指定type属性为SOCK_STREAM来使用TCP套接字。由于一台主机可能拥有多个IP地址,而且很有
可能会配置多个不同的服务,所以作为服务器端的程序,需要在创建套接字对象后将其绑定到指定的IP地址和
端口上。这里的端口并不是物理设备而是对IP地址的扩展,用于区分不同的服务,例如我们通常将HTTP服务
跟80端口绑定,而MySQL数据库服务默认绑定在3306端口,这样当服务器收到用户请求时就可以根据端口号
来确定到底用户请求的是HTTP服务器还是数据库服务器提供的服务。端口的取值范围是0~65535,而1024以
下的端口我们通常称之为“著名端口”(留给像FTP、HTTP、SMTP等“著名服务”使用的端口,有的地方也称之
为“周知端口”),自定义的服务通常不使用这些端口,除非自定义的是HTTP或FTP这样的著名服务。
"""
"""下面的代码实现了一个提供时间日期的服务器。"""
'''
from socket import socket,SOCK_STREAM,AF_INET
from datetime import datetime
def main():
# 1、创建套接字对象并指定使用哪种传输服务
# family = AF_INET -IPv4地址
# family = AF_INET6 -IPv6地址
# type = SOCK_STREAM -tcp套接字
# type = SOCK_DGRAM -udp套接字
# type = SOCK_RAW -原始套接字
server = socket(family=AF_INET,type=SOCK_STREAM)
# 2、绑定IP地址和端口号(端口用于区分不同的服务)
# 同一时间在同一个端口上只能绑定一个服务否则报错
server.bind(('127.0.0.1', 6789))
# 3、开启监听-监听客户端连接到服务器
# 参数512可以理解为链接队列的大小
server.listen(512)
print('服务器启动,开始监听...')
while True:
# 4、通过循环接收客户端的连接并做出相应的处理(提供服务)
# accept方法是一个阻塞方法如果没有客户端连接到服务器代码不会向下执行
# accept方法返回一个元组其中的第一个元素是客户端对象
# 第二个元素是连接到服务器的客户端的地址(由IP和端口两部分构成)
client,addr = server.accept()
print(str(addr) + '连接到了服务器.')
# 5、发送数据
client.send(str(datetime.now()).encode('utf-8'))
# 6、断开连接
client.close()
main()
'''
"""
当然我们也可以通过Python的程序来实现TCP客户端的功能,相较于实现服务器程序,实现客户端程序就简单多了,代码如下所示。
"""
'''
from socket import socket
def main():
# 1、创建套接字对象默认使用ipv4和tcp协议
client = socket()
# 2、连接到服务器(需要制定ip地址和端口)
client.connect(('127.0.0.1',6789))
# 3、从服务器接受数据
print(client.recv(1024).decode('utf-8'))
client.close()
main()
'''
# 多线程服务器
"""
需要注意的是,上面的服务器并没有使用多线程或者异步I/O的处理方式,这也就意味着当服务器与一个客户
端处于通信状态时,其他的客户端只能排队等待。很显然,这样的服务器并不能满足我们的需求,我们需要的
服务器是能够同时接纳和处理多个用户请求的。下面我们来设计一个使用多线程技术处理多个用户请求的服务
器,该服务器会向连接到服务器的客户端发送一张图片。
"""
'''
# 服务器端代码
from socket import socket,SOCK_STREAM,AF_INET
from base64 import b64encode
from json import dumps
from threading import Thread
def main():
# 自定义线程类
class FileTransferHandler(Thread):
def __init__(self,cclient):
super().__init__()
self.cclient = client
def run(self):
my_dict = {}
my_dict['filename'] = 'timg.jpg'
# json是纯文本不能携带二进制数据
# 所以图片的二进制数据要处理成base64编码
my_dict['filedata'] = data
# 通过dumps函数将字典处理成json字符串
json_str = dumps(my_dict)
# 发送json字符串
self.cclient.send(json_str.encode('utf-8'))
self.cclient.close()
# 1、创建套接字对象并指定使用哪种传输服务
server = socket()
# 2、绑定ip地址和端口(区分不同服务)
server.bind(('127.0.0.1',5566))
# 3、开启监听 --监听客户端连接到服务器
server.listen(512)
print('服务器启动,开始监听...')
with open('timg.jpg', 'rb') as f:
# 将二进制数据处理成base64在解码成字符串
data = b64encode(f.read()).decode('utf-8')
while True:
client,addr = server.accept()
# 启动一个线程来处理客户端的请求
FileTransferHandler(client).start()
if __name__ == '__main__':
main()
# 客户端代码
from socket import socket
from json import loads
from base64 import b64decode
def main():
client = socket()
client.connect(('127.0.0.1', 5566))
# 定义一个保存二进制数据的对象
in_data = bytes()
# 由于不知道服务器发送的数据由多大,每次接收1024字节
data = client.recv(1024)
while data:
# 将收到的数据拼接起来
in_data += data
data = client.recv(1024)
# 将收到的二进制数据解码成json字符串并转换为字典
# loads函数的作用就是将json字符串转换成字典对象
my_dict = loads(in_data.decode('utf-8'))
filename = my_dict['filename']
filedata = my_dict['filedata'].encode('utf-8')
with open('副本'+filename, 'wb') as f:
# 将base64格式的数据解码成二进制数据写入文件
f.write(b64decode(filedata))
print('图片已保存.')
if __name__ == '__main__':
main()
'''
"""
在这个案例中,我们使用了JSON作为数据传输的格式(通过JSON格式对传输的数据进行了序列化和反序列
的操作),但是JSON并不能携带二进制数据,因此对图片的二进制数据进行了Base64编码的处理。Base64是
一种用64个字符表示所有二进制数据的编码方式,通过将二进制数据每6位一组的方式重新组织,刚好可以使
用0~9的数字、大小写字母以及“+”和“/”总共64个字符表示从000000到111111的64种状态。
"""
# UDP套接字
"""
传输层除了有可靠的传输协议TCP之外,还有一种非常轻便的传输协议叫做用户数据报协议,简称UDP。TCP
和UDP都是提供端到端传输服务的协议,二者的差别就如同打电话和发短信的区别,后者不对传输的可靠性和
可达性做出任何承诺从而避免了TCP中握手和重传的开销,所以在强调性能和而不是数据完整性的场景中(例
如传输网络音视频数据),UDP可能是更好的选择。可能大家会注意到一个现象,就是在观看网络视频时,有
时会出现卡顿,有时会出现花屏,这无非就是部分数据传丢或传错造成的。在Python中也可以使用UDP套接字
来创建网络应用,对此我们不进行赘述,有兴趣的读者可以自行研究。
"""
# 网络应用开发
# 发送电子邮件
"""
在即时通信软件如此发达的今天,电子邮件仍然是互联网上使用最为广泛的应用之一,公司向应聘者发出录用
通知、网站向用户发送一个激活账号的链接、银行向客户推广它们的理财产品等几乎都是通过电子邮件来完成
的,而这些任务应该都是由程序自动完成的。
就像我们可以用HTTP(超文本传输协议)来访问一个网站一样,发送邮件要使用SMTP(简单邮件传输协
议),SMTP也是一个建立在TCP(传输控制协议)提供的可靠数据传输服务的基础上的应用级协议,它规定
了邮件的发送者如何跟发送邮件的服务器进行通信的细节,而Python中的smtplib模块将这些操作简化成了几
个简单的函数。
"""
"""下面的代码演示了如何在Python发送邮件。"""
'''
from smtplib import SMTP
from email.header import Header
from email.mime.text import MIMEText
def main():
sender = '<EMAIL>'
receivers = ['<EMAIL>']
message = MIMEText('用python发送邮件的实例代码.', 'plain','utf-8')
message['From'] = Header('王大锤','utf-8')
message['To'] = Header('王朝阳','utf-8')
message['Subject'] = Header('示例代码实验邮件','utf-8')
smtper = SMTP('smtp.qq.com')
smtper.login(sender, 'hfmrcdivpxayejhb')
smtper.sendmail(sender,receivers,message.as_string())
print('邮件发送完成')
main()
'''
# 发送短信
"""
发送短信也是项目中常见的功能,网站的注册码、验证码、营销信息基本上都是通过短信来发送给用户的。在
下面的代码中我们使用了互亿无线短信平台(该平台为注册用户提供了50条免费短信以及常用开发语言发送短
信的demo,可以登录该网站并在用户自服务页面中对短信进行配置)提供的API接口实现了发送短信的服务,
当然国内的短信平台很多,读者可以根据自己的需要进行选择(通常会考虑费用预算、短信达到率、使用的难
易程度等指标),如果需要在商业项目中使用短信服务建议购买短信平台提供的套餐服务。
"""
'''
import urllib.parse
import http.client
import json
def main():
host = "106.ihuyi.com"
sms_send_uri = "/webservice/sms.php?method=Submit"
params = urllib.parse.urlencode({'account':'C75138010','password':'<PASSWORD>','content':'您的验证码是:1234。请不要把验证码泄露给其他人。','mobile':'18729537656','format':'json'})
print(params)
headers = {'Content-type': 'application/x-www-form-urlencoded', 'Accept': 'text/plain'}
conn = http.client.HTTPConnection(host,port=80,timeout=30)
conn.request('POST',sms_send_uri,params,headers)
response = conn.getresponse()
response_str = response.read()
jsonstr = response_str.decode('utf-8')
print(json.loads(jsonstr))
conn.close()
'''
main()
<file_sep># 图形和办公文档处理
"""
用程序来处理图像和办公文档经常出现在实际开发中,Python的标准库中虽然没有直接支持这些操作的模块,
但我们可以通过Python生态圈中的第三方模块来完成这些操作。
用pillow操作图象
Pillow是由从著名的Python图像处理库PIL发展出来的一个分支,通过Pillow可以实现图像压缩和
图像处理等各
Pillow中最为重要的是Image类,读取和处理图像都要通过这个类来完成。
"""
'''
from PIL import Image
def main():
image = Image.open('./timg.jpg')
print(image.format,image.size,image.mode)
image.show()
main()
'''
# 1、裁剪图片
'''
from PIL import Image
image = Image.open('timg.jpg')
rect = 80,20,310,360
image.crop(rect).show()
'''
# 2、生成缩略图
'''
from PIL import Image
image = Image.open('timg.jpg')
size = 128,128
image.thumbnail(size)
image.show()
'''
# 3、缩放和黏贴图片
'''
from PIL import Image
image1 = Image.open('timg.jpg')
image2 = Image.open('猪妹.jpg')
rect = 100,20,200,260
pikaqiu_head = image2.crop(rect)
width,height = pikaqiu_head.size
image1.paste(pikaqiu_head.resize((int(width/1.5), int(height/1.5))),(172,40))
image1.show()
'''
# 4、旋转和翻转
'''
from PIL import Image
image = Image.open('猪妹.jpg')
image.rotate(180).show()
image.transpose(Image.FLIP_LEFT_RIGHT).show()
'''
# 5、操作像素
'''
from PIL import Image
image = Image.open('timg.jpg')
for x in range(80,310):
for y in range(20,360):
image.putpixel((x,y),(128,128,128))
image.show()
'''
# 6、滤镜效果
'''
from PIL import Image,ImageFilter
image = Image.open('timg.jpg')
image.filter(ImageFilter.CONTOUR).show() # 这里把图片变黑白了
'''
# 处理Excel电子表格
"""
Python的openpyxl模块让我们可以在Python程序中读取和修改Excel电子表格,当然实际工作中,我们可能会
用LibreOffice Calc和OpenOffice Calc来处理Excel的电子表格文件,这就意味着openpyxl模块也能处理来自这
些软件生成的电子表格。关于openpyxl的使用手册和使用文档可以查看它的官方文档。
链接:https://openpyxl.readthedocs.io/en/stable/#
"""
# 处理Word文档
"""
利用python-docx模块,Pytho 可以创建和修改Word文档,当然这里的Word文档不仅仅是指通过微软的
Office软件创建的扩展名为docx的文档,LibreOffice Writer和OpenOffice Writer都是免费的字处理软件。
"""
# 处理PDF文档
"""
PDF是Portable Document Format的缩写,使用.pdf作为文件扩展名。接下来我们就研究一下如何通过Python
实现从PDF读取文本内容和从已有的文档生成新的PDF文件。
"""
<file_sep># 字符串和正则表达式
# 使用正则表达式
"""
在编写处理字符串的程序或网页时,经常会有查找符合某些复杂规则的字符串的需要,正则表达式就是用于描述
这些规则的工具,换句话正则表达式是一种工具,它定义了字符串的匹配模式(检查一个字符串是否有跟某种模式
匹配的部分或者从一个字符串中将与模式匹配的部分提取出来或者替换掉)。
如果你在Windows操作系统中使用过文件查找并且在指定文件名时使用过通配符(*和?),
那么正则表达式也是与之类似的用来进行文本匹配的工具,只不过比起通配符正则表达
式更强大,它能更精确地描述你的需求(当然你付出的代价是书写一个正则表达式比打出一个通配
符要复杂得多,要知道任何给你带来好处的东西都是有代价的,就如同学习一门编程语言一样),
比如你可以编写一个正则表达式,用来查找所有以0开头,后面跟着2-3个数字,然后是一个连字
号“-”,最后是7或8位数字的字符串(像028-12345678或0813-7654321),这不就是国内的座机号
码吗。最初计算机是为了做数学运算而诞生的,处理的信息基本上都是数值,而今天我们在日常工
作中处理的信息基本上都是文本数据,我们希望计算机能够识别和处理符合某些模式的文本,正则
表达式就显得非常重要了。今天几乎所有的编程语言都提供了对正则表达式操作的支持,Python通
过标准库中的re模块来支持正则表达式操作。
我们可以考虑下面一个问题:我们从某个地方(可能是一个文本文件,也可能是网络上的一则新
闻)获得了一个字符串,希望在字符串中找出手机号和座机号。当然我们可以设定手机号是11位的
数字(注意并不是随机的11位数字,因为你没有见过“25012345678”这样的手机号吧)而座机号跟
上一段中描述的模式相同,如果不使用正则表达式要完成这个任务就会很麻烦。
关于正则表达式的相关知识,大家可以阅读一篇非常有名的博客叫《正则表达式30分钟入门教程》,
读完这篇文章后你就可以看懂下面的表格,这是我们对正则表达式中的一些基本符号进行的扼要总结。
不会查文档把
链接:https://github.com/jackfrued/Python-100-Days/blob/master/Day01-15/12.%E5%AD%97%E7%AC%A6%E4%B8%B2%E5%92%8C%E6%AD%A3%E5%88%99%E8%A1%A8%E8%BE%BE%E5%BC%8F.md
"""
# python对正则表达式的支持
"""
Python提供了re模块来支持正则表达式相关操作,下面是re模块中的核心函数。
函数 说明
compile(pattern, flags=0) 编译正则表达式返回正则表达式对象
match(pattern, string, flags=0) 用正则表达式匹配字符串 成功返回匹配对象 否则返回None
search(pattern, string, flags=0) 搜索字符串中第一次出现正则表达式的模式 成功返回匹配对象 否则返回None
split(pattern, string, maxsplit=0, flags=0) 用正则表达式指定的模式分隔符拆分字符串 返回列表
sub(pattern, repl, string, count=0, flags=0) 用指定的字符串替换原字符串中与正则表达式匹配的模式 可以用count指定替换的次数
fullmatch(pattern, string, flags=0) match 函数的完全匹配(从字符串开头到结尾)版本
findall(pattern, string, flags=0) 查找字符串所有与正则表达式匹配的模式 返回字符串的列表
finditer(pattern, string, flags=0) 查找字符串所有与正则表达式匹配的模式 返回一个迭代器
purge() 清除隐式编译的正则表达式的缓存
re.I / re.IGNORECASE 忽略大小写匹配标记
re.M / re.MULTILINE 多行匹配标记
说明: 上面提到的re模块中的这些函数,实际开发中也可以用正则表达式对象的方法替代对这些函数
的使用,如果一个正则表达式需要重复的使用,那么先通过compile函数编译正则表达式并创建出
正则表达式对象无疑是更为明智的选择。
"""
# 例子1:验证输入用户名和qq号是否有效并给出对应的提示信息
"""
验证输入的用户名和qq号是否有效并给出对应的提示信息
要求:用户名必须由字母,数字或下划线构成且长度在6-20和字符之间,qq号是5-12的数字4
且首位不为0
"""
'''
import re
def main():
username = input('请输入用户名:')
qq = input('请输入qq号:')
# match 函数的第一个参数是正则表达式字符串或正则表达式对象
# 第二个参数是要跟正则表达式做匹配的字符串对象
m1 = re.match(r'^[0-9a-zA-Z_]{6,20}$',username)
if not m1:
print('请输入有效的用户名.')
m2 = re.match(r'^[1-9]\d{4,11}$',qq)
if not m2:
print('请输入有效的qq号.')
if m1 and m2:
print('你输入的信息有效的!')
main()
提示: 上面在书写正则表达式时使用了“原始字符串”的写法(在字符串前面加上了r),
所谓“原始字符串”就是字符串中的每个字符都是它原始的意义,说得更直接一点就是字符串中没有
所谓的转义字符啦。
'''
# 例子2:从一段文字内提取出国内手机号码
'''
import re
def main():
# 创建正则表达式对象 使用了前瞻和回顾来保证手机号前后不应该出现数字
pattern = re.compile(r'(?<=\D)1[34578]\d{9}(?=\D)')
sentence =
重要的事情说8130123456789遍,我的手机号是13512346789这个靓号,
不是15600998765,也是110或119,王大锤的手机号才是15600998765。
# 查找所有匹配并保存到一个列表中
mylist = re.findall(pattern, sentence)
print(mylist)
print('--------华丽的分隔线--------')
# 通过迭代器取出匹配对象并获得匹配的内容
for temp in pattern.finditer(sentence):
print(temp.group())
print('--------华丽的分隔线--------')
# 通过search函数指定搜索位置找出所有匹配
m = pattern.search(sentence)
while m:
print(m.group())
# m = pattern.search(sentence, m.end())
if __name__ == '__main__':
main()
'''
# 例子3:替换字符串中的不良内容
'''
import re
def main():
sentence = '你丫是傻叉吗? 我操你大爷的.Fuck you.'
purified = re.sub('[操肏艹]|fuck|shit|傻[比屄逼叉缺吊屌]|煞笔','*',sentence,flags=re.IGNORECASE)
print(purified)
main()
'''
# 拆分长字符串
'''
import re
def main():
poem = '窗前明月光,疑是地上霜、举头望明月,低头思故乡。'
sentence_list = re.split(r'[,。.]',poem)
while '' in sentence_list:
sentence_list.remove('')
print(sentence_list)
main()
'''
"""
后话
如果要从事爬虫类应用的开发,那么正则表达式一定是一个非常好的助手,因为它可以帮助我们迅速
的从网页代码中发现某种我们指定的模式并提取出我们需要的信息,当然对于初学者来收,
要编写一个正确的适当的正则表达式可能并不是一件容易的事情(当然有些常用的正则表达式可以
直接在网上找找),所以实际开发爬虫应用的时候,有很多人会选择Beautiful Soup或Lxml来进行匹配
和信息的提取,前者简单方便但是性能较差,后者既好用性能也好,但是安装稍嫌麻烦,这
些内容我们会在后期的爬虫专题中为大家介绍。
"""
<file_sep>import socket
# 1 买手机
phone = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
# 2 插手机卡
phone.bind(('127.0.0.1', 9094)) # bind传入的是一个元祖
# 3 开机
phone.listen(5) # 代表最大挂起的链接数
# 4 等电话
print("start....")
conn, client_addr = phone.accept()
print(client_addr)
# 5 收发消息
while True: # 通信循环
data = conn.recv(1024) # 1 单位: bytes 2 1024代表最大接收1024个字节
print('客户端数据', data)
conn.send(data.upper())
conn.close()
phone.close()
<file_sep>import socket
import subprocess
import struct
# 1 买手机
phone = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
phone.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1)
# 2 插手机卡
phone.bind(('127.0.0.1', 9094)) # bind传入的是一个元祖
# 3 开机
phone.listen(5) # 代表最大挂起的链接数
# 4 等电话
print("start....")
while True: # 链接循环
conn, client_addr = phone.accept()
print(client_addr)
# 5 收发消息
while True: # 通信循环
# 客户端单方面断开链接,会导致服务端死循环
try:
# 1 接收命令
cmd = conn.recv(8096) # 1 单位: bytes 2 1024代表最大接收1024个字节
if not cmd: # 适用于Linux系统
break
# 2 执行命令, 拿到结果
obj = subprocess.Popen(cmd.decode('utf-8'), shell=True,
stdout=subprocess.PIPE,
stderr=subprocess.PIPE)
stdout = obj.stdout.read()
stderr = obj.stderr.read()
# 3 把命令的结果返回给客户端
# 第一步: 制作固定长度的报头
total_size = len(stdout) + len(stderr)
header = struct.pack('i', total_size)
# 第二步:把报头 (固定长度) 发送给客户端
conn.send(header)
# 第三步:在发真实的数据
conn.send(stdout)
conn.send(stderr)
except ConnectionResetError: #使用于windows系统
break
conn.close()
phone.close()
<file_sep>import socket
import subprocess
# 1 买手机
phone = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
phone.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1)
# 2 插手机卡
phone.bind(('127.0.0.1', 9094)) # bind传入的是一个元祖
# 3 开机
phone.listen(5) # 代表最大挂起的链接数
# 4 等电话
print("start....")
while True: # 链接循环
conn, client_addr = phone.accept()
print(client_addr)
# 5 收发消息
while True: # 通信循环
# 客户端单方面断开链接,会导致服务端死循环
try:
# 1 接收命令
cmd = conn.recv(1024) # 1 单位: bytes 2 1024代表最大接收1024个字节
if not cmd: # 适用于Linux系统
break
# 2 执行命令, 拿到结果
obj = subprocess.Popen(cmd.decode('utf-8'), shell=True,
stdout=subprocess.PIPE,
stderr=subprocess.PIPE)
stdout = obj.stdout.read()
stderr = obj.stderr.read()
# 3 把命令的结果返回给客户端
print(len(stdout+stderr))
conn.send(stdout+stderr) # + 是一个可以优化的点
except ConnectionResetError: #使用于windows系统
break
conn.close()
phone.close()
<file_sep>import sys
import pygame
from bullet import Bullet
from alien import Alien
from time import sleep
def check_keydown_events(event,ai_settings, screen, ship, bullets):
"""响应按键"""
if event.key == pygame.K_RIGHT:
ship.moving_right = True
elif event.key == pygame.K_LEFT:
ship.moving_left = True
elif event.key == pygame.K_SPACE:
fire_bullet(ai_settings, screen, ship, bullets)
elif event.key == pygame.K_q:
sys.exit()
def fire_bullet(ai_settings, screen,ship,bullets):
"""如果没有到达限制,就发射一颗子弹"""
#创建新子弹,并将其加入编组bullets中
if len(bullets) < ai_settings.bullet_allowed:
new_bullet = Bullet(ai_settings, screen, ship)
bullets.add(new_bullet)
def check_keyup_events(event, ship):
"""响应松开"""
if event.key == pygame.K_RIGHT:
ship.moving_right = False
elif event.key == pygame.K_LEFT:
ship.moving_left = False
def check_events(ai_settings, screen, ship, bullets):
"""响应按键和鼠标事件"""
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
elif event.type == pygame.KEYDOWN:
check_keydown_events(event,ai_settings, screen, ship, bullets)
elif event.type == pygame.KEYUP:
check_keyup_events(event, ship)
def update_screen(ai_settings, screen,stats, ship,aliens, bullets):
""""更新屏幕上的图像,并切换到新屏幕"""
#每次循环时都会重新绘制屏幕
screen.fill(ai_settings.bg_color)
#在飞船和外星人后面重绘所有子弹
for bullet in bullets.sprites():
bullet.draw_bullet()
ship.blitme()
aliens.draw(screen)
#如果游戏处于非活动状态, 就绘制Play按钮
#if not stats.game_active:
#play_button.draw_button()
#让最近绘制的屏幕可见
pygame.display.flip()
def check_bullet_alien_collisions(ai_settings, screen, ship, aliens, bullets):
"""相应子弹和外星人的碰撞"""
#删除发生碰撞的子弹和外星人
collections = pygame.sprite.groupcollide(bullets, aliens, True, True)
if len(aliens) == 0:
bullets.empty()
ai_settings.increase_speed()
creat_fleet(ai_settings, screen, ship, aliens)
"""(要模拟能够穿行到屏幕顶端的高能子弹 —— 消灭它击中的每个外星人,
可将第一个布尔实参设置为 False ,并让第二个布尔实参为 True 。
这样被击中的外星人将消失,但所有的子弹都始终有效,直到抵达屏幕顶端后消失。)"""
def update_bullets(ai_settings, screen, ship, aliens, bullets):
"""更新子弹位置,并删除已经消失的子弹"""
#更新子弹位置
bullets.update()
#删除已消失子弹位置
for bullet in bullets.copy():
if bullet.rect.bottom <= 0:
bullets.remove(bullet)
#print(len(bullets))
#检查是否有子弹击中了外星人
#如果是这样,就删除相应的子弹和外星人
check_bullet_alien_collisions(ai_settings, screen, ship, aliens, bullets)
def get_number_aliens_x(ai_settings, alien_width):
"""并计算一行可以容纳多少个外星人"""
available_space_x = ai_settings.screen_width -2*alien_width
number_aliens_x = int(available_space_x /(2*alien_width))
return number_aliens_x
def get_number_rows(ai_settings, ship_height, alien_height):
"""计算屏幕可以容纳多少个外星人"""
available_space_y = (ai_settings.screen_height - (3*alien_height)-ship_height)
numbers_rows = int(available_space_y /(2*alien_height))
return numbers_rows
def creat_alien(ai_settings, screen, aliens, alien_number, row_number):
"""创建一个外星人并将其加入当前行"""
alien = Alien(ai_settings, screen)
alien_width = alien.rect.width
alien.x = alien_width + 2*alien_width*alien_number
alien.rect.x = alien.x
alien.rect.y = alien.rect.height + 2*alien.rect.height*row_number
aliens.add(alien)
def creat_fleet(ai_settings, screen, ship, aliens):
"""创建外星人群"""
#创建一个外星人,并计算一行可以容纳多少个外星人
alien = Alien(ai_settings, screen)
number_aliens_x = get_number_aliens_x(ai_settings, alien.rect.width)
number_rows = get_number_rows(ai_settings, ship.rect.height,alien.rect.height)
#创建第一行外星人
for row_number in range(number_rows):
for alien_number in range(number_aliens_x):
creat_alien(ai_settings, screen, aliens, alien_number, row_number)
def check_fleet_edges(ai_settings, aliens):
"""有外星人到达边缘是采取相应措施"""
for alien in aliens.sprites():
if alien.check_edges():
change_fleet_direction(ai_settings, aliens)
break
def change_fleet_direction(ai_settings, aliens):
"""将整群外星人下移,并改变他们的方向"""
for alien in aliens.sprites():
alien.rect.y += ai_settings.fleet_drop_speed
ai_settings.fleet_direction *= -1
def ship_hit(ai_settings, stats, screen, ship, aliens, bullets):
"""相应被外星人撞到的飞船"""
if stats.ships_left > 0:
#将ship_left减1
stats.ships_left -= 1
#清空外星人列表和子弹列表
aliens.empty()
bullets.empty()
#创建一群新的外星人, 并将飞船放到屏幕低端中央
creat_fleet(ai_settings, screen, ship, aliens)
ship.center_ship()
#暂停
sleep(0.5)
else:
stats.game_active = False
def check_aliens_bottom(ai_settings, stats, screen, ship, aliens, bullets):
"""检查是否有外星人到达了屏幕底端"""
screen_rect = screen.get_rect()
for alien in aliens.sprites():
if alien.rect.bottom >= screen_rect.bottom:
#像飞船被撞到一样处理
ship_hit(ai_settings, stats, screen, ship, aliens, bullets)
break
def update_aliens(ai_settings,stats, screen, ship, aliens, bullets):
"""检查是否有外星人位于屏幕边缘, 更新外星人群中所有外星人的位置"""
check_fleet_edges(ai_settings,aliens)
aliens.update()
#检测外星人和飞船之间的碰撞
if pygame.sprite.spritecollideany(ship, aliens):
ship_hit(ai_settings, stats, screen, ship, aliens, bullets)
#检查是否有外星人到达屏幕底端
check_aliens_bottom(ai_settings, stats, screen, ship, aliens, bullets)
<file_sep># 面向对象编程基础
"""
活在当下的程序员应该都听过“面向对象编程”一词,也经常有人问能不能用一句话解释下什么是
“面向对象编程”,我们来看比较正式的说法。
把一组数据和处理他们的方法组成对象,把相同行为的对象归纳为类,通过类的封装,隐藏
内部细节,通过继承实现类的特化和泛化,通过多态实现基于对象类型的动态分配。
————————————————————————————————————————————————————————————————————————
封装:不管你是土鳖还是土豪,不管你中午吃的是窝头还是鲍鱼,你的下水都在你的肚皮里,别
人看不到你中午吃了啥,除非你自己说给他们听(或者画给他们看)
继承:刚说了,你个土鳖/豪,你们全家都是土豪/鳖。冰冻三尺非一日之寒,你有今天,必定可以
从你爸爸爷爷那里追根溯源。正所谓虎父无犬子,正恩同学那么狠,他爹正日就不是什么善茬,更甭说
他爷爷。
多态:哲学家说过,世上不会有两个一模一样的双胞胎。及及时你从你父亲那里继承来的土鳖/土豪气质
也完全不可能是从一个模子里刻出来的,总会有些差别。比如你爸爸喜欢蹲在门前面吃面,你喜欢骑
在村口的歪脖子树上吃,或者反过来。当然,也可能令尊喜欢吃鲍鱼时旁边有几个艺校小女生喝酒
唱歌助兴,你可能喜欢弄个街舞乐队来吹拉弹唱。
————————————————————————————————————————————————————————————————————————
之前我们说过“程序是指令的集合”,我们在程序中书写的语句在执行时会变成一条或多条指令然后由
CPU去执行。当然为了简化程序的设计,我们引入了函数的概念,把相对独立且经常重复使用的代码
放置到函数中,在需要使用这些功能的时候只要调用函数即可;如果一个函数的功能过于复杂和臃肿
我们又可以进一步将函数继续切分为子函数来降低系统的复杂性。但是说了这么多,不知道大家
是否发现,所谓编程就是程序员按照计算机的工作方式控制计算机完成各种任务。但是,计算机的
工作方式与正常人类的思维模式是不同的,如果编程就必须得抛弃人类正常的思维方式去迎合计算机
编程的乐趣就少了很多,“每个人都应该学习编程”这样的豪言壮语就只能说说而已。当然,这些还不
是最重要的,最重要的是当我们需要开发一个复杂的系统时,代码的复杂性会让开发和维护工作都变
得举步维艰,所以在上世纪60年代末期,“软件危机”、“软件工程”等一系列的概念开始在行业中出现。
当然,程序员圈子内的人都知道,现实中并没有解决上面所说的这些问题的“银弹”,真正让软件
开发者看到希望的是上世纪70年代诞生的Smalltalk编程语言中引入的面向对象的编程思想
(面向对象编程的雏形可以追溯到更早期的Simula语言)。按照这种编程理念,
程序中的数据和操作数据的函数是一个逻辑上的整体,我们称之为“对象”,而我们解决问题的方式
就是创建出需要的对象并向对象发出各种各样的消息,多个对象的协同工作最终可以让我们构造出
复杂的系统来解决现实中的问题。
说明: 当然面向对象也不是解决软件开发中所有问题的最后的“银弹”,所以今天的高级程序
设计语言几乎都提供了对多种编程范式的支持,Python也不例外。
———————————————————————————————————————————————————————————————————————
"""
# 类和对象
"""
简单的说,类是对象的蓝图和模板,而对象是类的实例。从这句话我们可以看出,类是抽象的概念
而对象是具体的东西。在面向对象编程的世界中,一切皆为对象,对象都有属性和行为,每个对象
都是独一无二的,而且对象是独一无二的,而且对象一定属于某个类(型)。当我们把一大堆拥有共同
特征的对象的静态特征(属性)和动态特征(行为)都抽取出来,就可以定义出一个叫做"类的东西"。
"""
# 定义类
'''
"""
在python中使用 class 关键字定义类,然后在类中通过函数来定义方法,这样就可以将对象的
动态特征描述出来。
"""
class Student(object):
# __init__是一个特殊方法用于在创建对象时进行初始化操作
# 通过这个方法我们可以为学生对象绑定nam和age两个属性
def __init__(self,name,age):
self.name = name
self.age = age
def study(self,course_name):
print('%s正在学习%s.'%(self.name, course_name))
# PEP 8要求标识符的名字用全小写,多个单词时下划线连接
# 但是很多程序员和公司更倾向与使用驼峰命名法
def watch_av(self):
if self.age < 18:
print('%s只能观看《熊出没》.' % self.name)
else:
print('%s正在观看到岛国爱情片.' % self.name)
# 说明:写在类中的函数,我们通常称之为(对象的方法),这些方法就是对象可以接受到的消息
'''
# 创建和使用对象
'''
def main():
# 创建学生对象并且指定姓名和年龄
stu1 = Student('王朝阳',22)
# 给对象发study消息
stu1.study('python程序设计')
# 给对象发watch_av消息
stu1.watch_av()
stu2 = Student('王大锤',15)
stu2.study('思想品德')
stu2.watch_av()
main()
'''
# 访问可见性的问题
'''
"""
对于上面的代码,有C++、Java、C#等编程经验的程序员可能会问,我们给Student对象绑定
的name和age属性到底具有怎样的访问权限(也称为可见性)。因为在很多面向对象编程语言中,
我们通常会将对象的属性设置为私有的(private)或受保护的(protected),
简单的说就是不允许外界访问,而对象的方法通常都是公开的(public),因为公开的方法就是
对象能够接受的消息。在Python中,属性和方法的访问权限只有两种,也就是公开的和私有的,
如果希望属性是私有的,在给属性命名时可以用两个下划线作为开头,下面的代码可以验证这一点。
"""
class Test:
def __init__(self,foo):
self.__foo = foo
def __bar(self):
print(self.__foo)
print('__bar')
def main():
test = Test('hello')
test.__bar() # AttributeError: 'Test' object has no attribute '__bar'
print(test.__foo) # AttributeError: 'Test' object has no attribute '__foo'
main()
'''
"""
但是,Python并没有从语法上严格保证私有属性或方法的私密性,它只是给私有的属性和方法换了
一个名字来“妨碍”对它们的访问,事实上如果你知道更换名字的规则仍然可以访问到它们,
下面的代码就可以验证这一点。之所以这样设定,可以用这样一句名言加以解释,
就是“We are all consenting adults here”。因为绝大多数程序员都认为开放比封闭要好,
而且程序员要自己为自己的行为负责。
"""
"""
class Test:
def __init__(self,foo):
self.__foo = foo
def __bar(self):
print(self.__foo)
print('__bar')
def main():
test = Test('hello')
test._Test__bar()
print(test._Test__foo)
main()
"""
"""
在实际开发中,我们并不建议将属性设置为私有的,因为这样会导致子类无法访问。所以大多数python
程序员会遵循一种命名习惯就是让属性以单下划线开头来表示属性是受保护的,本类之外的代码在
访问这样的属性时应该保持慎重。这种做法不是语法上的规则,单下划线的属性和方法外界仍然是可以
访问的,所以更多的时候它是一种暗示。
"""
# 面向对象的支柱
"""
面向对象有三大支柱:封装,继承和多态。我自己对封装的理解是“隐藏一切可以隐藏的实现细节,
只向外界暴露(提供)简单的编程接口”。我们在类中定义的方法其实就是把数据和对数据的
操作封装起来了,在我们创建了对象之后,只需要给对象发送一个消息(调用方法)就可以执行方法
中的代码,也就是说我们只需要知道方法的名字和传入的参数(方法的外部视图),而不需要知道
方法内部的实现细节(方法的内部视图)。
"""
"""——————————————————————————————————————————————————————————————————"""
# 练习1:定义一个类描述数字时钟
'''
import time
import os
class Clock(object):
"""数字时钟"""
def __init__(self,hour=0,minute=0,second=0):
"""初始化方法
:param hour:时
:param minute:分
:param second: 秒
"""
self._hour = hour
self._minute = minute
self._second = second
def run(self):
"""走字"""
self._second += 1
if self._second == 60:
self.second = 0
self._minute += 1
if self._minute == 60:
self.minute = 0
self._hour += 1
if self._hour == 24:
self._hour = 0
def show(self):
"""显示时间"""
return '%02d:%02d:%02d' % (self._hour,self._minute,self._second)
def main():
clock = Clock(23,58,00)
while True:
print(clock.show())
time.sleep(1)
clock.run()
main()
'''
# 练习2:定义一个类描述平面上的并提供移动点和计算到另外一个点的距离的方法
'''
from math import sqrt
class Point(object):
def __init__(self,x=0,y=0):
"""初始化方法
:param x: 横坐标
:param y: 纵坐标
"""
self.x = x
self.y = y
def move_to(self,x,y):
"""移动到指定位置
:param x: 新的横坐标
:param x: 新的纵坐标
"""
self.x = x
self.y = y
def move_by(self,dx,dy):
"""移动到指定增量
:param dx: 横坐标的增量
:param dy: 纵坐标的增量
"""
self.x += dx
self.y += dy
def distance_to(self,other):
"""计算与另外一个点的距离
:param otner: 另一个点
"""
dx = self.x - other.x
dy = self.y - other.y
return sqrt(dx**2 + dy**2)
def __str__(self):
return '(%s,%s)' % (str(self.x),str(self.y))
def main():
p1 = Point(3,5)
p2 = Point()
print(p1)
print(p2)
p2.move_by(-1,2)
print(p2)
print(p1.distance_to(p2))
main()
'''
<file_sep># Python语言进阶
# 1、数据结构与算法
"""
算法:解决问题的方法和步骤
评价算法的好坏:渐进时间复杂度和空间复杂度。
渐进时间复杂度的大O标记:
- 常量时间复杂度 - 布隆过滤器 / 哈希存储
- 对数时间复杂度 - 折半查找(二分查找)
- 线性时间复杂度 - 顺序查找 / 桶排序
- 对数线性时间复杂度 - 高级排序算法(归并排序、快速排序)
- 平方时间复杂度 - 简单排序算法(选择排序、插入排序、冒泡排序)
- 立方时间复杂度 - Floyd算法 / 矩阵乘法运算
- 几何级数时间复杂度 - 汉诺塔
- 阶乘时间复杂度 - 旅行经销商问题 - NP
"""
"""排序算法(选择,冒泡,归并)和查找算法(顺序和折半)"""
"""简单选择排序"""
'''
def select_sort(origin_items,comp=lambda x,y: x < y):
""""每次都去剩余的部分找最小的往前放"""
items = origin_items[:]
for i in range(len(items)-1):
min_index = i
for j in range(i+1, len(items)):
if comp(items[j],items[min_index]):
min_index = j
items[i],items[min_index] = items[min_index],items[i]
return items
def main():
a = [9,8,7,6,5,4,3,2,1]
b = select_sort(a)
print(a)
print(b)
'''
"""高质量冒泡排序(搅拌排序)"""
'''
def bubble_sort(origin_items, comp=lambda x,y: x>y):
items = origin_items[:]
for i in range(len(items)-1):
swapped = False
for j in range(i, len(items)-1-i):
if comp(items[j], items[j+1]):
items[j],items[j+1] = items[j+1],items[j]
swapped = True
if swapped:
swapped = False
for j in range(len(items)-2-i, i,-1):
if comp(items[j-1],items[j]):
items[j],items[j-1] = items[j-1],items[j]
swapped = True
if not swapped:
break
return items
import datetime
from random import randint
def main():
a = []
for i in range(1,10000):
a.append(randint(1,1000))
start = datetime.datetime.now()
b = select_sort(a)
b = bubble_sort(a)
end = datetime.datetime.now()
print(end-start)
main()
'''
"""归并排序(分治法)"""
'''
def merge_sort(items,comp=lambda x,y : x >= y):
if len(items) < 2:
return items[:]
mid = len(items) // 2
left = merge_sort(items[:mid],comp)
right = merge_sort(items[mid:],comp)
return merge(left,right,comp)
def merge(items1,items2,comp):
items = []
index1,index2 = 0,0
while index1 < len(items1) and index2 < len(items2):
if comp(items1[index1],items2[index2]):
items.append(items1[index1])
index1 += 1
else:
items.append(items2[index2])
index2 += 1
items += items1[index1:]
items += items2[index2:]
return items
def main():
l = [4,3,2,1]
ll = merge_sort(l,lambda x,y : x <= y)
print(ll)
main()
'''
"""顺序查找"""
'''
def seq_search(items,key):
for index,item in enumerate(items):
if item == key:
return index
return -1
'''
"""折半查找"""
'''
def bin_search(items,key):
start,end = 0,len(items)-1
while start <= end:
mid = (start + end) // 2
if key > items[mid]:
start = mid + 1
elif key < items[mid]:
end = mid - 1
else:
return mid
return -1
'''
"""使用生成式(推导式)语法"""
'''
prices = {
'AAPL': 191.88,
'GOOG': 1186.96,
'IBM': 149.24,
'ORCL': 48.44,
'ACN': 166.89,
'FB': 208.09,
'SYMC': 21.29
}
# 用股票价格大于100元的股票构造一个新的字典
prices2 = {key: value for key,value in prices.items() if value > 100}
print(prices2)
"""说明:生成式(推导式)可以用来生成列表,集合和字典"""
'''
"""嵌套的列表"""
'''
names= ['关羽','张飞','赵云','马超','黄忠']
courses = ['语文','数学','英语']
"""录入五个学生三门课的成绩"""
sorces = [[None] * len(courses) for _ in range(len(names))]
# print(sores)
for row,name in enumerate(names):
for col,course in enumerate(courses):
sorces[row][col] = float(input(f'请输入{name}的{course}成绩:'))
print(sorces)
'''
"""heapq,itertools等的用法"""
"""
从列表中找出最大的或最小的N个元素
堆结构(大根堆,小根堆)
"""
'''
import heapq
list1 = [34, 25, 12, 99, 87, 63, 58, 78, 88, 92]
list2 = [
{'name': 'IBM', 'shares': 100, 'price': 91.1},
{'name': 'AAPL', 'shares': 50, 'price': 543.22},
{'name': 'FB', 'shares': 200, 'price': 21.09},
{'name': 'HPQ', 'shares': 35, 'price': 31.75},
{'name': 'YHOO', 'shares': 45, 'price': 16.35},
{'name': 'ACME', 'shares': 75, 'price': 115.65}
]
print(heapq.nlargest(3,list1))
print(heapq.nsmallest(3,list1))
print(heapq.nlargest(2,list2,key=lambda x: x['price']))
print(heapq.nsmallest(2,list2,key=lambda x: x['shares']))
'''
"""迭代工具-排列/组合/笛卡尔积"""
'''
import itertools
print(list(itertools.permutations('ABCD')))
print(list(itertools.combinations('ABCDE',3)))
print(list(itertools.product('ABCD','123')))
'''
"""collections模块下的工具类"""
'''
from collections import Counter
words = [
'look', 'into', 'my', 'eyes', 'look', 'into', 'my', 'eyes',
'the', 'eyes', 'the', 'eyes', 'the', 'eyes', 'not', 'around',
'the', 'eyes', "don't", 'look', 'around', 'the', 'eyes',
'look', 'into', 'my', 'eyes', "you're", 'under'
]
counter = Counter(words)
print(counter.most_common(3))
# [('eyes', 8), ('the', 5), ('look', 4)]
'''
"""常用算法"""
"""
1、穷举法-又又称暴力破解法。对所有的可能性进行验证,直到找到正确答案
2、贪婪发-在对问题求解时,总是做出在当前看来最好的选择,不追求最优解,快速找到满意解
3、分治法-把一个复杂的问题分成两个或更多的相同或相似的子问题,在把子问题分成更小的子问题
直到可以直接求解的程度,最后将子问题的解进行合并得到原问题的解
4、回溯法-回溯法又称试探法,按选优条件向前搜索,当搜索到某一步发现原先选择并不优或达不到
目标时,就退一步重新选择
5、动态规划-基本思想是将带求解问题分成若干个子问题,先求解并保存这些子问题的解,避免产生
大量的重复计算
"""
"""穷举法例子"""
'''
# 公鸡5元一只 母鸡3元一只 小鸡1元三只
# 用100元买100只鸡 问公鸡/母鸡/小鸡各多少只
for x in range(20):
for y in range(33):
z = 100-x-y
if 5*x + 3*y + z//3 == 100 and z%3 == 0:
print(x,y,z)
'''
'''
# A、B、C、D、E五人在某天夜里合伙捕鱼 最后疲惫不堪各自睡觉
# 第二天A第一个醒来 他将鱼分为5份 扔掉多余的1条 拿走自己的一份
# B第二个醒来 也将鱼分为5份 扔掉多余的1条 拿走自己的一份
# 然后C、D、E依次醒来也按同样的方式分鱼 问他们至少捕了多少条鱼
fish = 6
while True:
total = fish
enough = True
for _ in range(5):
if(total-1)%5 == 0:
total = (total-1)//5*4
else:
enough = False
break
if enough:
print(fish)
break
fish += 5
'''
"""贪婪法例子"""
"""
假设小偷有一个背包,最多能装20公斤赃物,他闯入一户人家,发现如下表所示的物品。很显然,
他不能把所有物品都装入背包,所以确定拿走那些物品,留下哪些
名称 价格(美元) 重量(kg)
电脑 200 20
收音机 20 4
钟 175 10
花瓶 50 2
书 10 1
油画 90 9
______________________________________________________________________
贪婪法在求解问题时,总是做出在当前看来最好的选择,不追求最优解,快速找到满意解,。
"""
"""
class Thing(object):
# 物品
def __init__(self,name,price,weight):
self.name = name
self.price = price
self.weight = weight
@property
def value(self):
# 价格重量比
return self.price / self.weight
def input_thing():
# 输入物品信息
name_str,price_str,weight_str = input().split()
return name_str,int(price_str),int(weight_str)
def main():
# 主函数
max_weight,num_of_things = map(int, input().split())
all_things = []
for _ in range(num_of_things):
all_things.append(Thing(*input_thing()))
all_things.sort(key=lambda x: x.value,reverse=True)
total_weight = 0
total_price = 0
for thing in all_things:
if total_weight + thing.weight <= max_weight:
print(f'小偷拿走了{thing.name}')
total_weight += thing.weight
total_price += thing.price
print(f'总价值:{total_price}美元')
main()
"""
"""分治法例子:快速排序"""
"""
快速排序 - 选择枢轴元素进行划分,左边都比枢轴小,右边都比枢轴大
"""
"""
def quick_sort(origin_items,comp=lambda x,y: x <= y):
items = origin_items[:]
_quick_sort(items,0,len(items)-1,comp)
return items
def _quick_sort(items,start,end,comp):
if start < end:
pos = _partition(items,start,end,comp)
_quick_sort(items,start,pos-1,comp)
_quick_sort(items,pos+1,end,comp)
def _partition(items,start,end,comp):
pivot = items[end]
i = start -1
for j in range(start,end):
if comp(items[j],pivot):
i += 1
items[i],items[j] = items[j],items[i]
items[i+1],items[end] = items[end],items[i+1]
return i+1
def main():
import random
l = []
for _ in range(1,1000001):
l.append(random.randint(1,1000))
res = quick_sort(l)
print(res)
main()
"""
"""回溯法:骑士巡逻"""
"""
递归回溯法:叫试探法,按选优条件向前搜索,当搜索到某一步,发现原来选择并不优或达不到
"""
"""
import sys
import time
SIZE = 5
total = 0
def print_board(board):
for row in board:
for col in row:
print(str(col).center(4),end='')
print()
def patrol(board,row,col,step=1):
if row >= 0 and row < SIZE and col >= 0 and col < SIZE and board[row][col] == 0:
board[row][col] = step
if step == SIZE*SIZE:
global total
total += 1
print(f'第{total}种走法')
print_board(board)
patrol(board,row-2,col-2,step+1)
patrol(board,row-1,col-2,step+1)
patrol(board,row+1,col-2,step+1)
patrol(board,row+2,col-1,step+1)
patrol(board,row+2,col+1,step+1)
patrol(board,row+1,col+2,step+1)
patrol(board,row-1,col+2,step+1)
patrol(board,row-2,col+1,step+1)
board[row][col] = 0
def main():
board = [[0] * SIZE for _ in range(SIZE)]
patrol(board,SIZE-1,SIZE-1)
main()
"""
"""动态规划例子1:斐波拉契数列(不使用动态规划将会是几何级数复杂度)"""
"""
动态规划 - 适用于有重叠子问题和最优子结构性质的问题
使用动态规划方法所耗费时间往往远少于朴素解法(用空间换时间)
"""
"""
def fib(num,temp={}):
# 用递归计算Fibonacci数
if num in (1,2):
return 1
try:
return temp[num]
except KeyError:
temp[num] = fib(num-1) + fib(num-2)
return temp[num]
def main():
print(fib(905))
print(len(str(fib(750))))
main()
"""
"""动态规划例子2:子列表元素之和的最大值(可使用动态规划避免二重循环)"""
"""
def main():
items = list(map(int,input().split()))
size = len(items)
overall,partial = {},{}
overall[size-1] = partial[size-1] = items[size-1]
for i in range(size-2,-1,-1):
partial[i] = max(items[i],partial[i+1]+items[i])
overall[i] = max(partial[i],overall[i+1])
print(overall[0])
main()
"""
# 2、函数的使用方法
"""
1、将函数视为“一等公民”
函数可以赋值给变量
函数可以作为函数的参数
函数可以作为函数的返回值
2、高阶函数的用法(filter,map以及它们的替代品)
"""
"""
filter() 函数用于过滤序列,过滤掉不符合条件的元素,返回由符合条件元素组成的新列表。
该接收两个参数,第一个为函数,第二个为序列,序列的每个元素作为参数传递给函数进行判,
然后返回 True 或 False,最后将返回 True 的元素放到新列表中。
"""
"""
items1 = list(map(lambda x: x ** 2, filter(lambda x:x % 2,range(1,10))))
print(items1)
items2 = [x ** 2 for x in range(1,10) if x % 2] # 奇数%2余1,
print(items2)
"""
"""
3、位置参数、可变参数、关键字参数、命名关键字参数
4、参数的元信息(代码可读性问题)
5、匿名函数和内联函数的用法(lambda函数)
6、闭包和作用域问题
python中搜索变量的LEGB顺序(Local--> Embeded--> Global--> Built-in)
global 和 nonlocal 关键字的作用
global:声明或定义全局变量(要么使用现有的全局作用域的变量,要么定义一个变量放到全局作用域)
nonllocal:声明使用嵌套作用域的变量(嵌套作用域必须存在该变量,否则报错)
7、装饰器函数(使用装饰器和取消装饰器)
"""
# 例子:输出函数执行时间的装饰器
from functools import wraps
from time import time
def main(num):
while num:
print("hello world")
num = num - 1
def record_time(func):
"""自定义装饰函数的装饰器"""
@wraps(func)
def wrapper(*args,**kwargs):
start = time()
result = func(*args,**kwargs)
print(f'{func.__name__}:{time()-start}秒')
return result
return wrapper
record_time(main(1000))
<file_sep># python初始 和 语言元素
"""
Python的优缺点
Python的优点很多,简单的可以总结为以下几点。
简单和明确,做一件事只有一种方法。
学习曲线低,跟其他很多语言相比,Python更容易上手。
开放源代码,拥有强大的社区和生态圈。
解释型语言,天生具有平台可移植性。
支持两种主流的编程范式(面向对象编程和函数式编程)都提供了支持。
可扩展性和可嵌入性,可以调用C/C++代码,也可以在C/C++中调用Python。
代码规范程度高,可读性强,适合有代码洁癖和强迫症的人群。
————————————————————————————————————————————————————————————————————————————
Python的缺点主要集中在以下几点。
执行效率稍低,因此计算密集型任务可以由C/C++编写。
代码无法加密,但是现在的公司很多都不是卖软件而是卖服务,这个问题会被淡化。
在开发时可以选择的框架太多(如Web框架就有100多个),有选择的地方就有错误。
Python的应用领域
目前Python在云基础设施、DevOps、网络爬虫开发、数据分析挖掘、机器学习等领域都有着广泛的应用,
因此也产生了Web后端开发、数据接口开发、自动化运维、自动化测试、科学计算和可视化、
数据分析、量化交易、机器人开发、图像识别和处理等一系列的职位。
"""
'''
import sys
print(sys.version_info)
print(sys.version)
'''
'''
import turtle
turtle.pensize(4)
turtle.pencolor('red')
turtle.forward(100)
turtle.right(90)
turtle.forward(100)
turtle.right(90)
turtle.forward(100)
turtle.right(90)
turtle.forward(100)
turtle.mainloop()
'''
"""——————————————————————————————————————————————————————————————————————————————————————"""
# 指令和程序
"""
计算机的硬件系统通常由五大部件构成,包括:运算器、控制器、存储器、输入设备和输出设备。
其中,运算器和控制器放在一起就是我们通常所说的中央处理器,它的功能是执行各种运算和控制指令以及处理计算机软件中的数据。
“冯·诺依曼结构”有两个关键点,一是指出要将存储设备与中央处理器分开,二是提出了将数据以二进制方式编码。
"""
# 变量和类型
"""
在程序设计中,变量是一种存储数据的载体。计算机中的变量是实际存在的数据或者说是存储器中存储数据的一块内存空间,
变量的值可以被读取和修改,这是所有计算和控制的基础。
计算机能处理的数据有很多种类型,除了数值之外还可以处理文本、图形、音频、视频等各种各样的数据,那么不同的数据就需要定义不同的存储类型
整型:Python中可以处理任意大小的整数(Python 2.x中有int和long两种类型的整数,但这种区分对Python来说意义不大,
因此在Python 3.x中整数只有int这一种了),而且支持二进制(如0b100,换算成十进制是4)、
八进制(如0o100,换算成十进制是64)、十进制(100)和十六进制(0x100,换算成十进制是256)的表示法。
浮点型:浮点数也就是小数,之所以称为浮点数,是因为按照科学记数法表示时,
一个浮点数的小数点位置是可变的,浮点数除了数学写法(如123.456)之外还支持科学计数法(如1.23456e2)。
字符串型:字符串是以单引号或双引号括起来的任意文本,比如'hello'和"hello",
字符串还有原始字符串表示法、字节字符串表示法、Unicode字符串表示法,
而且可以书写成多行的形式(用三个单引号或三个双引号开头,三个单引号或三个双引号结尾)。
布尔型:布尔值只有True、False两种值,要么是True,要么是False,在Python中,可以直接用True、
False表示布尔值(请注意大小写),也可以通过布尔运算计算出来(例如3 < 5会产生布尔值True,而2 == 1会产生布尔值False)。
复数型:形如3+5j,跟数学上的复数表示一样,唯一不同的是虚部的i换成了j
"""
# 变量命名
"""
硬性规则:
变量名由字母(广义的Unicode字符,不包括特殊字符)、数字和下划线构成,数字不能开头。
大小写敏感(大写的a和小写的A是两个不同的变量)。
不要跟关键字(有特殊含义的单词,后面会讲到)和系统保留字(如函数、模块等的名字)冲突。
PEP 8要求:
用小写字母拼写,多个单词用下划线连接。
受保护的实例属性用单个下划线开头(后面会讲到)。
私有的实例属性用两个下划线开头(后面会讲到)。
"""
'''
a = 321
b = 123
print(a + b)
print(a - b)
print(a * b)
print(a / b)
print(a // b)
print(a % b)
print(a ** b)
'''
"""
使用input函数输入
使用int()进行类型转换
用占位符格式化输出的字符串
"""
'''
a = int(input('a = '))
b = int(input('b = '))
print('%d + %d = %d' % (a, b, a + b))
print('%d // %d = %d' % (a, b, a // b))
print('%d ** %d = %d' % (a, b, a ** b))
'''
"""
使用type()检查变量的类型
"""
'''
a = 100
b = 12.345
c = 1 + 5j
d = 'hello world'
e = True
print(type(a))
print(type(int(b))
print(type(c))
print(type(d))
print(type(e))
'''
"""
在对变量类型进行转换时可以使用Python的内置函数
(准确的说下面列出的并不是真正意义上的函数,而是后面我们要讲到的创建对象的构造方法)。
int():将一个数值或字符串转换成整数,可以指定进制。
float():将一个字符串转换成浮点数。
str():将指定的对象转换成字符串形式,可以指定编码。
chr():将整数转换成该编码对应的字符串(一个字符)。
ord():将字符串(一个字符)转换成对应的编码(整数)。
"""
'''
a = 5
b = 10
a /= b
print('a = ', a)
'''
'''
flag1 = 3 > 2
flag2 = 2 < 1
flag3 = flag1 and flag2
flag4 = flag1 or flag2
flag5 = not flag1
print("flag1 = ", flag1)
print("flag3 = ", flag3)
print("flag4 = " , flag4)
print("flag5 = ", flag5)
print(flag1 is True)
print(flag2 is not False)
'''
'''
# 华氏温度转为摄氏温度
f = float(input('请输入华氏温度:'))
c = (f-32)/1.8
print('%.1f华氏度 = %.1f摄氏度' %(f, c))
'''
'''
# 输入半径计算园的周长和面积
import math
while True:
radius = float(input('请输入园的半径:'))
perimetr = 2*math.pi*radius
area = math.pi*radius*radius
print('周长:%.2f' % perimetr)
print('面积:%.2f' % area)
'''
'''
# 输入的年份判断是不是闰年
year = int(input('请输入年份:'))
is_leap = (year%4==0 and year%100!=0 or year%400==0)
print(is_leap)
'''
<file_sep># 进程和线程
"""
今天我们使用的计算机早已进入多CPU或多核时代,而我们使用的操作系统都是支持“多任务”的操作系统,这
使得我们可以同时运行多个程序,也可以将一个程序分解为若干个相对独立的子任务,让多个子任务并发的执
行,从而缩短程序的执行时间,同时也让用户获得更好的体验。因此在当下不管是用什么编程语言进行开发
实现让程序同时执行多个任务也就是常说的“并发编程”,应该是程序员必备技能之一。为此,我们需要先讨论
两个概念,一个叫进程,一个叫线程。
概念
进程就是操作系统中执行的一个程序,操作系统以进程为单位分配存储空间,每个进程都有自己的地址空间、
数据栈以及其他用于跟踪进程执行的辅助数据,操作系统管理所有进程的执行,为它们合理的分配资源。进程
可以通过fork或spawn的方式来创建新的进程来执行其他的任务,不过新的进程也有自己独立的内存空间,因
此必须通过进程间通信机制(IPC,Inter-Process Communication)来实现数据共享,具体的方式包括管道
信号、套接字、共享内存区等。
一个进程还可以拥有多个并发的执行线索,简单的说就是拥有多个可以获得CPU调度的执行单元,这就是所谓
的线程。由于线程在同一个进程下,它们可以共享相同的上下文,因此相对于进程而言,线程间的信息共享和
通信更加容易。当然在单核CPU系统中,真正的并发是不可能的,因为在某个时刻能够获得CPU的只有唯一的
一个线程,多个线程共享了CPU的执行时间。使用多线程实现并发编程为程序带来的好处是不言而喻的,最主
要的体现在提升程序的性能和改善用户体验,今天我们使用的软件几乎都用到了多线程技术,这一点可以利用
系统自带的进程监控工具(如macOS中的“活动监视器”、Windows中的“任务管理器”)来证实,如下图所示。
当然多线程也并不是没有坏处,站在其他进程的角度,多线程的程序对其他程序并不友好,因为它占用了更多
的CPU执行时间,导致其他程序无法获得足够的CPU执行时间;另一方面,站在开发者的角度,编写和调试多
线程的程序都对开发者有较高的要求,对于初学者来说更加困难。
Python既支持多进程又支持多线程,因此使用Python实现并发编程主要有3种方式:多进程、多线程、多进程+多线程。
"""
# python中的多进程
"""
Unix和Linux操作系统上提供了fork()系统调用来创建进程,调用fork()函数的是父进程,创建出的是子
进程,子进程是父进程的一个拷贝,但是子进程拥有自己的PID。fork()函数非常特殊它会返回两次,父进
程中可以通过fork()函数的返回值得到子进程的PID,而子进程中的返回值永远都是0。Python的os模块提
供了fork()函数。由于Windows系统没有fork()调用,因此要实现跨平台的多进程编程,可以使用
multiprocessing模块的Process类来创建子进程,而且该模块还提供了更高级的封装,例如批量启动进程的
进程池(Pool)、用于进程间通信的队列(Queue)和管道(Pipe)等。
"""
"""下面用一个下载文件的例子来说明使用多进程和不使用多进程到底有什么差别,先看看下面的代码。"""
'''
from random import randint
from time import time,sleep
def download_task(filename):
print('开始下载%s...' % filename)
time_to_download = randint(5,10)
sleep(time_to_download)
print('%s下载完成! 耗费了%d秒!' % (filename, time_to_download))
def main():
start = time()
download_task('Python从入门到放弃.pdf')
download_task('Peking Hot.avi')
end = time()
print('总共耗费了%.2f秒.' %(end-start))
main()
开始下载Python从入门到放弃.pdf...
Python从入门到放弃.pdf下载完成! 耗费了9秒!
开始下载Peking Hot.avi...
Peking Hot.avi下载完成! 耗费了10秒!
总共耗费了19.03秒
'''
"""
从上面的例子可以看出,如果程序中的代码只能按照顺序一点点往下执行,那么即使执行两个毫不相关的
下载任务,也需要等待一个文件下载完成后才能开始下一个下载任务,很显然着并不合理也没有效率。
接下来我们使用多进程的方式将两个下载任务放到不同的进程中,代码如下所示。
"""
'''
from multiprocessing import Process
from os import getpid
from random import randint
from time import time,sleep
def download_task(filename):
print('启动下载进程,进程号[%d].' % getpid())
print('开始下载%s...' % filename)
time_to_download = randint(5,10)
sleep(time_to_download)
print('%s下载完成! 耗费了%d秒' % (filename, time_to_download))
def main():
start = time()
p1 = Process(target=download_task,args=('Python从入门到住院.pdf', ))
p1.start()
p2 = Process(target=download_task, args=('Peking Hot.avi', ))
p2.start()
p1.join()
p2.join()
end = time()
print('总共耗费了%.2f秒.' % (end-start))
if __name__ == '__main__':
main()
'''
"""
在上面的代码中,我们通过Process类创建了进程对象,通过 target 参数我们传入一个函数表示
进程启动后要执行的代码,后面的ards是一个元祖,它代表了传递给函数的参数。Process对象的
start 方法用来启动进程,而join 方法表示等待进程执行结束。运行上面的代码可以明显发现两个
下载任务“同时”启动了,而且程序的执行时间将大大缩短,不再是两个任务的时间总和。
下面是程序的一次执行结果。
启动下载进程,进程号[6124].
开始下载Python从入门到住院.pdf...
启动下载进程,进程号[17756].
开始下载Peking Hot.avi...
Peking Hot.avi下载完成! 耗费了5秒
Python从入门到住院.pdf下载完成! 耗费了6秒
总共耗费了6.14秒.
我们也可以使用subprocess模块中的类和函数来创建和启动子进程,然后通过管道和子进程通信,
这些内容不作讲解,有兴趣的读者可以自己了解这些知识。接下来我们将重点放在如何实现两个进程间的通
我们启动两个进程,一个输出Ping,一个输出Pong,两个进程输出的Ping和Pong加起来一共10个。听起
来很简单吧,但是如果这样写可是错的哦。
"""
'''
from multiprocessing import Process
from time import sleep
counter = 0
def sub_task(string):
global counter
while counter < 10:
print(string,end='',flush=True)
counter += 1
sleep(0.01)
def main():
Process(target=sub_task,args=('Ping',)).start()
Process(target=sub_task,args=('Pong',)).start()
if __name__ == '__main__':
main()
'''
"""
看起来没毛病,但是最后的的结果是Ping和Pong各输出了10个,Why?当我们在程序中创建进程的时候,子进
程复制了父进程及其所有的数据结构,每个子进程有自己独立的内存空间,这也就意味着两个子进程中各有一
个counter变量,所以结果也就可想而知了。要解决这个问题比较简单的办法是使用multiprocessing模块中
的Queue类,它是可以被多个进程共享的队列,底层是通过管道和信号量(semaphore)机制来实现的,有
兴趣的读者可以自己尝试一下。
"""
# Python中的多线程
"""
在Python早期的版本中就引入了thread模块(现在名为_thread)来实现多线程编程,然而该模块
过于底层,而且很多功能没有提供,因此目前的多线程开推荐使用threading模块,该模块对多线程
编程提供了更好的面向对象的封装。我们把刚才下载文件的例子用多线程的方式来实现一遍。
"""
'''
from random import randint
from threading import Thread
from time import time,sleep
def download(filename):
print('开始下载%s...' % filename)
time_to_download = randint(5,10)
sleep(time_to_download)
print('%s下载完成! 耗费%d秒' % (filename, time_to_download))
def main():
start = time()
t1 = Thread(target=download,args=('Python从入门到放弃.pdf', ))
t1.start()
t2 = Thread(target=download,args=('Peking Hot.avi', ))
t2.start()
t1.join()
t2.join()
end = time()
print('总共耗耗费了%.3f秒' % (end-start))
if __name__ == '__main__':
main()
'''
"""
我们可以直接使用threading模块的 Thread 类来创建线程,但是我们之前讲过“继承”,我们可以
从已有的类创建新类,因此也可以通过继承 Thread 类的方式来创建自定义的线程类,然后在创建
线程对象并启动线程。
"""
'''
from random import randint
from threading import Thread
from time import time,sleep
class DownloadTask(Thread):
def __init__(self, filename):
super().__init__()
self._filename = filename
def run(self):
print('开始下载%s...' % self._filename)
time_to_download = randint(5,10)
sleep(time_to_download)
print('%s下载完成!耗费了%d秒' % (self._filename, time_to_download))
def main():
start = time()
t1 = DownloadTask('Python从入门到住院.pdf')
t1.start()
t2 = DownloadTask('Peking Hot.avi')
t2.start()
t1.join()
t2.join()
end = time()
print('总共耗费了%.2f秒.' % (end-start))
if __name__ == '__main__':
main()
'''
"""
因为多个线程可以共享进程的内存空间,因此要实现多个线程间的通信向对简单,大家想到的最直接的
方法就是设置一个全局变量,多个线程共享这个全局变量即可。但是当多个线程共享同一个变量(称之为“资源”)
的时候,很有可能发生不可控制的结果从而导致程序崩溃。如果一个资源被多个线程竞争使用,通常
称之为“临界资源”,对“临界资源”的访问需要加上保护,否则资源会处于“混乱”的状态。
面的例子演示了100个线程向同一个银行账户转账(转入1元钱)的场景,在这个例子中,
银行账户就是一个临界资源,在没有保护的情况下我们很有可能会得到错误的结果。
"""
'''
from time import sleep
from threading import Thread
class Account(object):
def __init__(self):
self._balance = 0
def deposit(self,money):
# 计算存款后的余额
new_balance = self._balance + money
# 模拟受理存款业务需要0.01秒的时间
sleep(0.01)
# 修改账户余额
self._balance = new_balance
@property
def balance(self):
return self._balance
class AddMoneyThread(Thread):
def __init__(self,account,money):
super().__init__()
self._account = account
self._money = money
def run(self):
self._account.deposit(self._money)
def main():
account = Account()
threads = []
# 创建100个存款的线程向同一个账户中存钱
for _ in range(100):
t =AddMoneyThread(account, 1)
threads.append(t)
t.start()
# 等所有存款的线程都执行完毕
for t in threads:
t.join()
print('账户余额为¥%d元' % account.balance)
if __name__ == '__main__':
main()
'''
"""
运行上面的程序,结果让人大跌眼镜,100个线程分别向账户中转入1元钱,结果居然远远小于100元。之所以
出现这种情况是因为我们没有对银行账户这个“临界资源”加以保护,多个线程同时向账户中存钱时,会一起执
行到new_balance = self._balance + money这行代码,多个线程得到的账户余额都是初始状态下的
0,所以都是0上面做了+1的操作,因此得到了错误的结果。在这种情况下,“锁”就可以派上用场了。我们
可以通过“锁”来保护“临界资源”,只有获得“锁”的线程才能访问“临界资源”,而其他没有得到“锁”的线程只能被
阻塞起来,直到获得“锁”的线程释放了“锁”,其他线程才有机会获得“锁”,进而访问被保护的“临界资源”。下面
的代码演示了如何使用“锁”来保护对银行账户的操作,从而获得正确的结果。
"""
'''
from time import sleep
from threading import Thread,Lock
class Account(object):
def __init__(self):
self._balance = 0
self._lock = Lock()
def deposit(self,money):
# 先获取锁才能执行后续的代码
self._lock.acquire()
try:
new_balance = self._balance + money
sleep(0.01)
self._balance = new_balance
finally:
# 在finally中执行释放锁的操作保证正常,异常锁都能释放
self._lock.release()
@property
def balance(self):
return self._balance
class AddMoneyThread(Thread):
def __init__(self,account,money):
super().__init__()
self._account = account
self._money = money
def run(self):
self._account.deposit(self._money)
def main():
account = Account()
threads = []
for _ in range(100):
t = AddMoneyThread(account,1)
threads.append(t)
t.start()
for t in threads:
t.join()
print('账户余额:¥%d元' % account.balance)
if __name__ == '__main__':
main()
'''
"""
比较遗憾的一件事情是Python的多线程并不能发挥CPU的多核特性,这一点只要启动几个执行死循环的线程就
可以得到证实了。之所以如此,是因为Python的解释器有一个“全局解释器锁”(GIL)的东西,任何线程执行
前必须先获得GIL锁,然后每执行100条字节码,解释器就自动释放GIL锁,让别的线程有机会执行,这是一个
历史遗留问题,但是即便如此,就如我们之前举的例子,使用多线程在提升执行效率和改善用户体验方面仍然
仍然是有积极意义的。
"""
# 多线程还是多进程
"""
无论是多进程还是多线程,只要数量一多,效率肯定上不去,为什么呢?我们打个比方,假设你不幸正在准备
中考,每天晚上需要做语文、数学、英语、物理、化学这5科的作业,每项作业耗时1小时。如果你先花1小时
做语文作业,做完了,再花1小时做数学作业,这样,依次全部做完,一共花5小时,这种方式称为单任务模
型。如果你打算切换到多任务模型,可以先做1分钟语文,再切换到数学作业,做1分钟,再切换到英语,以此
类推,只要切换速度足够快,这种方式就和单核CPU执行多任务是一样的了,以旁观者的角度来看,你就正在
同时写5科作业。
但是,切换作业是有代价的,比如从语文切到数学,要先收拾桌子上的语文书本、钢笔(这叫保存现场),然
后,打开数学课本、找出圆规直尺(这叫准备新环境),才能开始做数学作业。操作系统在切换进程或者线程
时也是一样的,它需要先保存当前执行的现场环境(CPU寄存器状态、内存页等),然后,把新任务的执行环
境准备好(恢复上次的寄存器状态,切换内存页等),才能开始执行。这个切换过程虽然很快,但是也需要耗
费时间。如果有几千个任务同时进行,操作系统可能就主要忙着切换任务,根本没有多少时间去执行任务了,
这种情况最常见的就是硬盘狂响,点窗口无反应,系统处于假死状态。所以,多任务一旦多到一个限度,反而
会使得系统性能急剧下降,最终导致所有任务都做不好。
是否采用多任务的第二个考虑是任务的类型,可以把任务分为计算密集型和I/O密集型。计算密集型任务的特
是要进行大量的计算,消耗CPU资源,比如对视频进行编码解码或者格式转换等等,这种任务全靠CPU的运
算能力,虽然也可以用多任务完成,但是任务越多,花在任务切换的时间就越多,CPU执行任务的效率就越
低。计算密集型任务由于主要消耗CPU资源,这类任务用Python这样的脚本语言去执行效率通常很低,最能胜
任这类任务的是C语言,我们之前提到了Python中有嵌入C/C++代码的机制。
除了计算密集型任务,其他的涉及到网络、存储介质I/O的任务都可以视为I/O密集型任务,这类任务的特点是
CPU消耗很少,任务的大部分时间都在等待I/O操作完成(因为I/O的速度远远低于CPU和内存的速度)。对于
I/O密集型任务,如果启动多任务,就可以减少I/O等待时间从而让CPU高效率的运转。有一大类的任务都属于
I/O密集型任务,这其中包括了我们很快会涉及到的网络应用和Web应用。
"""
# 单线程+异步I/O
"""
现代操作系统对I/O操作的改进中最为重要的就是支持异步I/O。如果充分利用操作系统提供的异步I/O支持,
就可以用单进程单线程模型来执行多任务,这种全新的模型称为事件驱动模型。Nginx就是支持异步I/O的Web
服务器,它在单核CPU上采用单进程模型就可以高效地支持多任务。在多核CPU上,可以运行多个进程(数量
与CPU核心数相同),充分利用多核CPU。用Node.js开发的服务器端程序也使用了这种工作模式,这也是当下
实现多任务编程的一种趋势。
在Python语言中,单线程+异步I/O的编程模型称为协程,有了协程的支持,就可以基于事件驱动编写高效的
多任务程序。协程最大的优势就是极高的执行效率,因为子程序切换不是线程切换,而是由程序自身控制,因
此,没有线程切换的开销。协程的第二个优势就是不需要多线程的锁机制,因为只有一个线程,也不存在同时
写变量冲突,在协程中控制共享资源不用加锁,只需要判断状态就好了,所以执行效率比多线程高很多。如果
想要充分利用CPU的多核特性,最简单的方法是多进程+协程,既充分利用多核,又充分发挥协程的高效率,
可获得极高的性能。关于这方面的内容,我稍后会做一个专题来进行讲解。
"""
# 应用案例
# 例子1:将耗时间的任务放到线程中以获得更好的体验
"""
如下所示的界面中,有“下载”和“关于”两个按钮,用休眠的方式模拟点击“下载”按钮会联网下载文件需要耗费
10秒的时间,如果不使用“多线程”,我们会发现,当点击“下载”按钮后整个程序的其他部分都被这个耗时间的
任务阻塞而无法执行了,这显然是非常糟糕的用户体验,代码如下所示。
"""
'''
import time
import tkinter
import tkinter.messagebox
def download():
# 模拟下载任务需要花费10秒的时间
time.sleep(10)
tkinter.messagebox.showinfo('提示','下载完成!')
def show_about():
tkinter.messagebox.showinfo('关于','作者:王朝阳(v1.0)')
def main():
top = tkinter.Tk()
top.title('单线程')
top.geometry('200x150')
top.wm_attributes('-topmost',True)
panel = tkinter.Frame(top)
button1 = tkinter.Button(panel,text='下载',command=download)
button1.pack(side='left')
button2 = tkinter.Button(panel,text='关于',command=show_about)
button2.pack(side='right')
panel.pack(side='bottom')
tkinter.mainloop()
main()
'''
"""
如果使用多线程将耗时间的任务放到一个独立的线程中执行,这样就不会因为执行耗时间的任务而阻塞了主线
程,修改后的代码如下所示。
"""
'''
import time
import tkinter
import tkinter.messagebox
from threading import Thread
def main():
class DownloadTaskHandler(Thread):
def run(self):
time.sleep(10)
tkinter.messagebox.showinfo('提示','下载完成')
# 启用下载按钮
button1.config(state=tkinter.NORMAL)
def download():
# 禁用下载按钮
button1.config(state=tkinter.DISABLED)
# 通过daemon参数将线程设置为守护线程(主程序退出就不在保留执行)
# 在线程中处理耗费时间的下载任务
DownloadTaskHandler(daemon=True).start()
def show_about():
tkinter.messagebox.showinfo('关于','作者:王朝阳(v.2.0)')
top = tkinter.Tk()
top.title('单线程')
top.geometry('300x200')
top.wm_attributes('-topmost',1)
panel = tkinter.Frame(top)
button1 = tkinter.Button(panel,text='下载',command=download)
button1.pack(side='left')
button2 = tkinter.Button(panel,text='关于',command=show_about)
button2.pack(side='right')
panel.pack(side='bottom')
tkinter.mainloop()
if __name__ == '__main__':
main()
'''
# 例子2:使用多进程对复杂任务进行“分而治之”
"""
我们来完成1~100000000求和的计算密集型任务,这个问题本身非常简单,有点循环的知识就能解决,
代码如下所示。
"""
'''
from time import time
def main():
total = 0
number_list = (x for x in range(1,100000001))
start = time()
for number in number_list:
total += number
print(total)
end = time()
print('Execution time: %3fs' % (end-start))
if __name__ == '__main__':
main()
'''
"""
在上面的代码中,我故意先去创建了一个列表容器然后填入了100000000个数,这一步其实是比较耗时间的,
所以为了公平起见,当我们将这个任务分解到8个进程中去执行的时候,我们暂时也不考虑列表切片操作花费
的时间,只是把做运算和合并运算结果的时间统计出来,代码如下所示。
"""
'''
from multiprocessing import Process,Queue
from random import randint
from time import time
def task_handler(curr_list, result_queue):
total = 0
for number in curr_list:
total += number_class
result_queue.put(total)
def main():
processes = []
number_list1 = (x for x in range(1,100000001))
number_list = list(number_list1)
# 这里会出现内存错误,应该是定义的列表过大
result_queue = Queue()
index = 0
# 启动8个线程将数据切片后进行运算
for _ in range(8):
p = Process(target=task_handler,args=(number_list[index:index + 12500000],result_queue))
index += 12500000
processes.append(p)
p.start()
# 开始记录所有进程执行完成花费的时间
start = time()
for p in processes:
p.join()
# 合并执行结果
total = 0
while not result_queue.empty():
total += result_queue.get()
print(total)
end = time()
print('Execution time:',(end-start),'s',sep='')
if __name__ == '__main__':
main()
'''
"""
比较两段代码的执行结果(在我目前使用的MacBook上,上面的代码需要大概6秒左右的时间,而下面的代码
只需要不到1秒的时间,再强调一次我们只是比较了运算的时间,不考虑列表创建及切片操作花费的时间),
使用多进程后由于获得了更多的CPU执行时间以及更好的利用了CPU的多核特性,明显的减少了程序的执行时
间,而且计算量越大效果越明显。当然,如果愿意还可以将多个进程部署在不同的计算机上,做成分布式进
程,具体的做法就是通过multiprocessing.managers模块中提供的管理器将Queue对象通过网络共享出来(注
册到网络上让其他计算机可以访问),这部分内容也留到爬虫的专题再进行讲解。
"""
<file_sep># 函数和模块的使用
# 上面的问题等同于将8个苹果分成四组每组至少一个苹果有多少种方案
# 求阶乘
'''
def factorial(num):
result = 1
for n in range(1,num + 1):
result *= n
return result
m = int(input('m = '))
n = int(input('n = '))
print(factorial(m) // factorial(n) // factorial(m-n))
'''
# 定义函数
"""
在Python中可以使用def关键字来定义函数,和变量一样每个函数也有一个响亮的名字,
而且命名规则跟变量的命名规则是一致的。在函数名后面的圆括号中可以放置传递给
函数的参数,这一点和数学上的函数非常相似,程序中函数的参数就相当于是数学上
说的函数的自变量,而函数执行完成后我们可以通过return关键字来返回一个值,
这相当于数学上说的函数的因变量。
"""
# 函数的参数
"""
在Python中,函数的参数可以有默认值,也支持使用可变参数,
所以Python并不需要像其他语言一样支持函数的重载,因为我们在定义一个函数
的时候可以让它有多种不同的使用方式,下面是两个小例子。
"""
# 摇筛子 param n:色子的个数 return: n颗色子点数之和
'''
from random import randint
def roll_dice(n=2):
total = 0
for _ in range(n):
total += randint(1, 6)
return total
def add(a=0, b=0, c=0):
return a + b + c
# 如果没有使用参数 使用默认值摇筛子
print(roll_dice())
# 摇三颗
print(roll_dice(3))
print(add())
print(add(1))
print(add(1, 2))
print(add(1, 2, 3))
#传递参数时可以不按照设定的顺序进行传递
print(add(c=50, a=100, b=200))
'''
# 可变参数
# 在参数名前面的*表示args是一个可变参数
# 即在调用add函数时可以传入0个或多个参数
'''
def add(*args):
total = 0
for val in args:
total += val
return total
print(add())
print(add(1))
print(add(1,2,3,4))
print(add(1,3,5,7,9))
'''
# 用模块管理函数
"""
因为我们会遇到命名冲突这种尴尬的情况。
最简单的场景就是在同一个.py文件中定义了两个同名函数,由于Python没有函数重载的概念,
那么后面的定义会覆盖之前的定义,也就意味着两个函数同名函数实际上只有一个是存在的。
def foo():
print('hello, world!')
def foo():
print('goodbye, world!')
# 下面的代码会输出什么呢?
foo()
当然上面的这种情况我们很容易就能避免,但是如果项目是由多人协作进行团队开发的时候,
团队中可能有多个程序员都定义了名为foo的函数,那么怎么解决这种命名冲突呢?
答案其实很简单,Python中每个文件就代表了一个模块(module),我们在不同的模块中可以
有同名的函数,在使用函数的时候我们通过import关键字导入指定的模块就可以区分到底要
使用的是哪个模块中的foo函数,代码如下所示。
module1.py
def foo():
print('hello, world!')
module2.py
def foo():
print('goodbye, world!')
test.py
from module1 import foo
# 输出hello, world!
foo()
from module2 import foo
# 输出goodbye, world!
foo()
也可以按照如下所示的方式来区分到底要使用哪一个foo函数。
test.py
import module1 as m1
import module2 as m2
m1.foo()
m2.foo()
但是如果将代码写成了下面的样子,那么程序中调用的是最后导入的那个foo,
因为后导入的foo覆盖了之前导入的foo。
test.py
from module1 import foo
from module2 import foo
# 输出goodbye, world!
foo()
test.py
from module2 import foo
from module1 import foo
# 输出hello, world!
foo()
需要说明的是,如果我们导入的模块除了定义函数之外还中有可以执行代码,
那么Python解释器在导入这个模块时就会执行这些代码,事实上我们可能并不希望如此,
因此如果我们在模块中编写了执行代码,最好是将这些执行代码放入如下所示的条件中
,这样的话除非直接运行该模块,if条件下的这些代码是不会执行的,
因为只有直接执行的模块的名字才是“__main__”。
module3.py
def foo():
pass
def bar():
pass
# __name__是Python中一个隐含的变量它代表了模块的名字
# 只有被Python解释器直接执行的模块的名字才是__main__
if __name__ == '__main__':
print('call foo()')
foo()
print('call bar()')
bar()
test.py
import module3
# 导入module3时 不会执行模块中if条件成立时的代码 因为模块的名字是module3而不是__main__
"""
# 练习
# 实现计算求最大公约数和最小公倍数的函数
'''
def gcd(x, y):
(x, y) = (y, x) if x > y else (x, y)
# 从x到0(左闭右开)->[x 0) 每次减1
for factor in range(x,0,-1):
if x % factor == 0 and y %factor == 0:
return factor
def lcm(x, y):
return x*y // gcd(x,y)
'''
# 实现判断一个数是不是回文数的函数
'''
def is_palindrome(num):
temp = num
total = 0
while temp > 0:
total = total * 10 + temp % 10
temp //= 10
return total == num
'''
# 实现判断一个数是不是素数
'''
def is_prime(num):
for factor in range(2,num):
if num % factor == 0:
return False
return True if num != 1 else False
'''
# 实现一个程序判断输入的正整数是不是回文素数
'''
if __name__ == '__main__':
num = int(input('请输入整数:'))
if is_palindrome(num) and is_prime(num):
print("%d是回文素数" % num)
'''
<file_sep>import socket
import time
# 1 买手机
client = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
# 2 拨号
client.connect(('127.0.0.1', 9094))
client.send('hello'.encode('utf-8'))
time.sleep(5)
client.send('world'.encode('utf-8'))
<file_sep># 分支结构
'''
username = input('请输入用户名:')
password = input('请输入口令:')
# 如果希望输入口令时,终端没有回显,可以使用getpass模块的getpass函数
# import getpass
# password = getpass.getpass('请输入口令:')
if username == 'admin' and password == '<PASSWORD>':
print('身份验证成功!')
else:
print('身份验证失败!')
'''
'''
import getpass
username = input('请输入用户名:')
password = getpass.getpass('请输入口令:')
if username == 'admin' and password == '<PASSWORD>':
print('身份验证成功!')
else:
print('身份验证失败!')
'''
'''
x = float(input('x = '))
if x > 1:
y = 3 * x - 5
elif x < -1:
y = x + 2
else:
y = 5 * x + 3
print('x = %.2f : y = %.2f' % (x, y))
'''
'''
x = float(input('x = '))
if x >= -1 and x <=1:
y = x + 2
else:
if x > 1:
y = 3 * x - 5
else:
5 * x + 3
print('x = %.2f, y = %.2f' %(x, y))
'''
"""
大家可以自己感受一下这两种写法到底是哪一种更好。
在之前我们提到的Python之禅中有这么一句话“Flat is better than nested.”,
之所以提出这个观点是因为嵌套结构的嵌套层次多了之后会严重的影响代码的可读性,
如果可以使用扁平化的结构就不要去用嵌套,因此之前的写法是更好的做法。
"""
# 练习1:英制单位和公制单位转换
'''
value = float(input('请输入长度:'))
unit = input('请输入单位:')
if unit == 'in' or unit == '英寸':
print('%f英寸 = %f厘米' %(value, value*2.54))
elif unit == 'cm' or unit == '厘米':
print('%d厘米 = %f英寸' %(value, value/2.54))
else:
print('请输入有效的单位')
'''
# 练习2:掷色子决定做什么
'''
from random import randint
face = randint(1,6)
if face == 1:
result = '唱歌'
elif face == 2:
result = '跳个舞'
elif face == 3:
result = '学狗叫'
elif face == 4:
result = '做俯卧撑'
elif face == 5:
result = '念绕口令'
else:
result = '讲笑话'
print(result)
'''
# 练习3:百分制成绩转等级
"""
百分制成绩转等级制成绩
90分以上 --> A
80分~89分 --> B
70分~79分 --> C
60分~69分 --> D
60分以下 --> E
Version: 0.1
Author: 骆昊
"""
'''
sorce = float(input('请输入成绩:'))
if sorce >= 90:
grade = 'A'
elif sorce >= 80:
grade = 'B'
elif sorce >= 70:
grade = 'C'
elif sorce >= 60:
grade = 'D'
else:
grade = 'E'
print('对应的等级是',grade)
'''
# 练习4:输入三条边如果能构成三角形就计算周长和面积
'''
import math
a = float(input('a = '))
b = float(input('b = '))
c = float(input('c = '))
if a + b > c and a + c > b and b + c > a:
print('周长:%f' %(a+b+c))
p = (a + b + c)/2
area = math.sqrt(p*(p-a)*(p-b)*(p-c))
print('面积:%f' %(area))
else:
print('不能构成三角形')
'''
# 练习5:个人所得税计算器
'''
salary = float(input('本月收入:'))
insurance = float(input('五险一金:'))
diff = salary - insurance - 3500
if diff <= 0:
rate = 0
deduction = 0
elif diff < 1500:
rate = 0.03
deduction = 0
elif diff < 4500:
rate = 0.01
deduction = 105
elif diff < 9000:
rate = 0.2
deduction = 555
elif diff < 35000:
rate = 0.25
deduction = 1005
elif deii < 55000:
rate = 0.3
deduction = 2755
elif diff < 80000:
rate = 0.35
deduction = 5500
else:
rate = 0.45
deduction = 13505
tax = abs(diff*rate - deduction)
print('个人所得税:%.2f' % tax)
print('实际到手收入:%.2f' % (diff + 3500-tax))
'''
<file_sep>import struct
'''
res = struct.pack('i', 128000000)
print(res, type(res), len(res))
# conn.recv(4)
obj = struct.unpack('i', res)
print(obj[0])
'''
# res = struct.pack('l', 12800000044443243442432)
# print(res, len(res))
<file_sep>import socket
import struct
# 1 买手机
phone = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
# 2 拨号
phone.connect(('127.0.0.1', 9094))
# 3 发、收消息
while True:
# 1 发命令
cmd = input('>> ').strip() # ls
if not cmd:
continue
phone.send(cmd.encode('utf-8'))
# 2 拿到命令的结果, 并打印
# 第一步:先拿到报头
header = phone.recv(4)
total_size = struct.unpack('i', header)[0]
# 第二步:从报头中解析出对真实数据的描述信息
# 第三步:接收真实的数据
recv_size = 0
recv_data=b''
while recv_size < total_size:
res= phone.recv(1024) # 1024是一个坑
recv_data += res
recv_size += len(res)
print(recv_data.decode('gbk'))
# 4 关闭
phone.close()
<file_sep>import socket
# 1 买手机
phone = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
# 2 拨号
phone.connect(('127.0.0.1', 9094))
# 3 发、收消息
while True:
# 1 发命令
cmd = input('>> ').strip() # ls
if not cmd:
continue
phone.send(cmd.encode('utf-8'))
# 2 拿到命令的结果, 并打印
data = phone.recv(1024) # 1024是一个坑
print(data.decode('gbk'))
# 4 关闭
phone.close()
<file_sep>import socket
import subprocess
import struct
import json
# 1 买手机
phone = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
phone.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1)
# 2 插手机卡
phone.bind(('127.0.0.1', 9094)) # bind传入的是一个元祖
# 3 开机
phone.listen(5) # 代表最大挂起的链接数
# 4 等电话
print("start....")
while True: # 链接循环
conn, client_addr = phone.accept()
print(client_addr)
# 5 收发消息
while True: # 通信循环
# 客户端单方面断开链接,会导致服务端死循环
try:
# 1 接收命令
cmd = conn.recv(8096) # 1 单位: bytes 2 1024代表最大接收1024个字节
if not cmd: # 适用于Linux系统
break
# 2 执行命令, 拿到结果
obj = subprocess.Popen(cmd.decode('utf-8'), shell=True,
stdout=subprocess.PIPE,
stderr=subprocess.PIPE)
stdout = obj.stdout.read()
stderr = obj.stderr.read()
# 3 把命令的结果返回给客户端
# 第一步: 制作固定长度的报头
header_dict = {
'filename' : 'a.txt',
'md5' : 'xxxfxx',
'total_size' : len(stdout) + len(stderr)
}
header_json = json.dumps(header_dict)
header_bytes = header_json.encode('utf-8')
# 第二步: 先发送报头的长度
conn.send(struct.pack('i', len(header_bytes)))
# 第三步:再发送报头
conn.send(header_bytes)
# 第四步:在发真实的数据
conn.send(stdout)
conn.send(stderr)
except ConnectionResetError: #使用于windows系统
break
conn.close()
phone.close()
<file_sep># 循环结构
"""
or-in循环
如果明确的知道循环执行的次数或者是要对一个容器进行迭代,那么我们推荐使用for-in循环,
"""
# 用for循环实现1-100求和
'''
sum = 0
for x in range(101):
sum += x
print(sum)
# 上面代码中的range类型,range可以用来产生一个不变的数值序列,而且这个序列通常都是用在循环中
# range(101) 可以产生一个0-100的整数序列
# range(1,100)可以产生一个1-99的整数序列
# range(1,100,2)可以产生一个1到99的奇数序列,其中的2是步长,即数值序列的增量
'''
# 用for循环实现1-100之间的偶数求和
'''
sum = 0
for x in range(2,101,2):
sum += x
print(sum)
'''
'''
sum = 0
for x in range(1,101):
if x % 2 == 0:
sum += x
print(sum)
'''
# while 循环
# 猜数字游戏
'''
import random
answer = random.randint(1,101)
counter = 0
while True:
counter += 1
number = int(input('请输入:'))
if number < answer:
print('大一点')
elif number > answer:
print('小一点')
else:
print('恭喜你猜对了!')
break
print('你总共猜了%f' % counter)
if counter > 7:
print('你的智商余额明显不足')
'''
# 输出乘法口诀表
'''
for i in range(1,10):
for j in range(1,i+1):
print('%d*%d=%d' %(i,j,i*j),end = '\t')
print()
'''
# 练习1:判断一个数是不是素数
'''
from math import sqrt
num = int(input('请输入一个整数:'))
end = int(sqrt(num))
is_prime = True
for x in range(2,end+1):
if num % x == 0:
is_prime = False
break
if is_prime and num != 1:
print('%d是素数' % num)
else:
print('%d不是素数' % num)
'''
# 练习2:输入两个整数,计算最大公约数和最小公倍数
'''
x = int(input('x = '))
y = int(input('y = '))
if x > y:
x,y = y,x
for factor in range(x,0,-1):
if x % factor == 0 and y % factor == 0:
print('%d和%d的最大公约数是%d' % (x, y, factor))
print('%d和%d的最小公倍数是%d' % (x, y, x * y//factor))
break
'''
# 练习3:打印三角形图案
"""
打印各种三角形图案
*
**
***
****
*****
*
**
***
****
*****
*
***
*****
*******
*********
Version: 0.1
Author: 骆昊
"""
'''
row = int(input('请输入行数:'))
for i in range(row):
for _ in range(i+1):
print('*',end='')
print()
for i in range(row):
for j in range(row):
if j < row -i - 1:
print(' ',end='')
else:
print('*', end='')
print()
for i in range(row):
for _ in range(row - i -1):
print(' ',end='')
for _ in range(2* i + 1):
print('*',end='')
print()
'''
<file_sep># python中的字符串、列表、元祖、集合、字典
'''
def main():
# 字符串操作
str1 = "hello, world!"
# 通过len函数计算字符串的长度
print(len(str1))
# 获取字符串首字母大写的拷贝
print(str1.capitalize())
# 获取字符串变大写后的拷贝
print(str1.upper())
# 在字符串中查找字串所在的位置
print(str1.find('or'))
print(str1.find('shit'))
# 与find类似但找不到字串时会引发异常
#print(str1.index('or'))
#print(str1.index('shit'))
# 检查字符串是否以指定的字符串开头
print(str1.startswith('He'))
print(str1.startswith('hel'))
# 检查字符串是否以指定字符串结尾
print(str1.endswith('!'))
# 将字符串以指定的宽度居中并在两侧填充指定的字符
print(str1.center(50,' '))
# 指定字符串以指定的宽度靠右放置左侧填充指定字符
print(str1.rjust(50,' '))
'''
'''
str2 = 'abc123456'
# 从字符串中取出指定位置的字符(下标运算)
print(str2[2]) # c
# 字符串切片(从指定的开始索引到指定的结束索引)
print(str2[2:5]) # c12
print(str2[2:]) # c123456
print(str2[2::2]) # c246
print(str2[::2]) # ac246
print(str2[::-1]) # 654321cba
print(str2[::-2]) # 642ca
print(str2[-3:-1]) # 45
# 检查字符串是否由数字构成
print(str2.isdigit()) # False
# 检查字符串是否以字母构成
print(str2.isalpha()) # False
# 检查字符串是否以数字和字母构成
print(str2.isalnum()) # True
'''
'''
str3 = ' <EMAIL>'
print(str3)
# 获得字符串修建左右两侧空格的拷贝
print(str3.strip())
'''
'''
# 列表及其操作
def main():
list1 = [1, 3, 5 ,7, 100]
print(list1)
list2 = ['hello'] * 5
print(list2)
# 计算列表长度
print(len(list1))
# 下标索引运算
print(list1[0])
print(list1[4])
# print(list1[5]) # IndexError: list index out of range
print(list1[-1])
print(list1[-3])
list1[2] = 300
print(list1)
# 添加元素
list1.append(200)
print(list1)
list1.insert(1,400) # 在1的位置插入400,把所有数据向后挪动
print(list1)
list1 += [1000, 2000]
# list1 = [] # python是一个边解释边运行的语言,后面定义的list1会把之前的覆盖,不会像C/C++的语言报错
print(list1)
# 删除元素
list1.remove(3) # 删除是如果元素在列表中不存在,会报异常
print(list1)
if 1234 in list1:
list1.remove(1234)
print(list1)
del list1[0]
print(list1)
# 清空列表元素
list1.clear()
print(list1)
'''
'''
和字符串一样,列表也可以做切片操作,通过切片操作,我们可以实现对列表的复制
或者将列表中的一部分取出来创建出新的列表
'''
'''
def main():
fruits = ['grape', 'apple', 'strawberry', 'waxberry']
# print(fruits)
fruits += ['pitaya', 'pear', 'mango']
# print(fruits)
# 循环遍历列表元素
for fruit in fruits:
print(fruit.title(), end=' ')
print()
# 列表切片
fruits2 = fruits[1:4]
print(fruits2)
fruit3 = fruits # 没有复制列表,只是创建了新的引用
# 可以通过完整的切片操作来复制列表
fruits3 = fruits[:]
print(fruits3)
fruits4 = fruits[-3:-1]
print(fruits4)
# 可以通过反向切片操作来获得翻转后的列表的拷贝
fruits5 = fruits[::-1]
print(fruits5)
'''
'''
# 下面的代码实现了对列表的排序操作
def main():
list1 = ['orange', 'apple', 'zoo', 'pear', 'blueberry']
list2 = sorted(list1)
print(list1)
print(list2)
# sorted函数返回列表排序后的拷贝不会修改传入的列表
# 函数的设计就应该像sorted一样尽可能不产生副作用
list3 = sorted(list1, reverse=True)
print(list3)
# 通过key关键字参数指定根据字符串长度进行排序,而不是默认的字母表顺序
list4 = sorted(list1, key=len)
print(list4)
# 给列表对象发出排序消息直接在列表对象上进行排序
list1.sort(reverse=True)
print(list1)
'''
# 使用列表的生成语法来创建列表
'''
import sys
def main():
f = [x for x in range(1,10)]
print(f)
f = [x + y for x in 'ABCDE' for y in '123456']
print(f)
# 使用列表的生成表达式语法创建列表容器
# 用这种语法创建列表之后元素已经准备就绪所以需要耗费较多的内存空间
f = [x ** 2 for x in range(1,1000)]
print(sys.getsizeof(f)) # 查看对象占用内存的字节数
print(f)
# 请注意下面的代码创建的不是一个列表而是一个生成器对象
# 通过生成器对象可以获取到数据,但它不占用额外的空间存储数据
# 每次需要数据的时候就通过内部的运算得到数据(需要花额外的时间)
f = (x ** 2 for x in range(1,1000))
print(sys.getsizeof(f)) # 相比生成器不占用存储数据的空间
print(f)
for val in f:
print(val,end=' ')
'''
'''
除了上面的生成器语法,python中还有另外一种定义生成器的方式,就是通过yield关键字
将一个普通函数改造成生成器函数。下面的代码演示了如何生成一个斐波那锲数列的生成器。
'''
'''
def fib(n):
a,b = 0,1
for _ in range(n):
a,b = b,a+b
yield a
def main():
for val in fib(20):
print(val)
'''
'''
def main1():
list1 = ['123',3, True]
print(list1)
'''
# 元祖
'''
python的元祖与列表类似,不同之处在于元祖的元素不能修改
顾名思义,我们把多个元素组合到一起就形成了一个元祖,所以它和列表一样可以保存多条数据。
'''
'''
def main():
# 定义元祖
t = ('王朝阳','23',True,'陕西西安')
print(t)
# 获取元祖中的元素
print(t[0])
print(t[3])
# 遍历元祖中的值
for member in t:
print(member)
# 重新给元祖赋值
# t[0] = '王大锤' # TypeError: 'tuple' object does not support item assignment
# 变量t重新引用了新的元祖,原来的元祖将被垃圾回收
t = ('王大锤',20,True, '广州深圳')
print(t)
# 将元祖转换成列表
person = list(t)
print(person)
# 列表是可以修改它的元素的
person[0] = '李小龙'
person[1] = 25
print(person)
# 将列表转化为元祖
fruits_list = ['apple', 'banana', 'orange']
fruits_tuple = tuple(fruits_list)
print(fruits_tuple)
'''
'''
这里有一个非常值得探讨的问题,我们已经有了列表这种数据结构,为什么还需要元祖这种类型呢
1、元祖中的元素是无法修改的,事实上我们在项目中,尤其是多线程环境中可能更喜欢使用的是那些
不变对象(一方面的因为对象状态不能更改,所以可以避免由此引起的不必要的程序错误,简单的
说就是一个不可变的对象要比一个可变的对象更加容易维护;另一方面是因为没有一个线程能够修改
不变对象的内部状态,一个不变对象自动就是线程安全的,这样就可以省掉处理同步化的开销。一个
不变对象可以方便的被共享访问)。所以结论就是:如果不需要对元素进行添加,删除,修改的时候,
可以考虑使用元祖,当然如果一个方法要返回多个值,使用元祖也是不错的选择.
2、元祖在创建时间和占用的空间上面都优于列表。我们可以使用sys模块的getsizeof函数来检查
存储同样的元素的元祖和列表各自占用了多少的内存空间。我们也可以在ipython中使用魔法指令
%timeit来分析创建同样内容的元祖和列表所花费的时间。
'''
'''
def main() :
import sys
tuple1 = (1,2,3,4,5,6) # 52
print(sys.getsizeof(tuple1))
list1 = [1,2,3,4,5,6] # 60
print(sys.getsizeof(list1))
'''
# 集合
# python中的集合跟数学上的集合上一致的,不允许有重复元素,而且可以进行交集、并集、差集的运算
'''
def main():
# 集合可以类比c++中的set理解,会对插入的数据进行排序+去重
set1 = {1,2,3,3,3,2}
print(set1)
print('lengrh =',len(set1))
set2 = set(range(1,10))
print(set2)
set1.add(4)
set1.add(5)
set2.update([11,12])
print(set1)
print(set2)
set2.discard(100) # 删除,没有不会抛异常
print(set2)
# remove的元素如果不存在会引发keyError
if 4 in set2:
set2.remove(4)
print(set2)
# 遍历集合容器
for elem in set2:
print(elem ** 2,end=' ')
print()
# 将元祖转换成集合
set3 = set((1,2,3,3,2,1))
print(set3.pop())
print(set3)
'''
'''
set1 = {1,2,3,3}
set2 = {1,2,3,4,5}
# 集合的交集、并集、差集、对称差运算
print(set1 & set2)
print(set1 | set2)
print(set1 - set2)
print(set1 ^ set2)
# 判断子集和超集
print(set1 <= set2)
print(set1 >= set2)
print(set3)
'''
# 字典
'''
def main():
sorces = {'小A':95, '小B':78, '小C':82}
print(sorces)
# 通过键可以获取字典中对应的值
print(sorces['小A'])
# 对字典进行遍历(遍历的其实是键在通过键取对应的值)
for elem in sorces:
print('%s ---> %d' % (elem,sorces[elem]))
# 更新字典中的元素
sorces['小B'] = 65
print(sorces)
sorces['小D'] = 71
print(sorces)
sorces.update(小E=67,小F=85)
print(sorces)
if '小G' in sorces:
print(sorces['小G'])
print(sorces.get('小G'))
# get方法也是通过键获取对应的的值,但是可以设置默认值
print(sorces.get('小G',False))
# 删除字典中的元素
print(sorces.popitem())
print(sorces.popitem())
print(sorces)
print(sorces.pop('小A',1000))
# 清空字典
sorces.clear()
print(sorces)
'''
########################################################################
# 练习1:在屏幕上显示跑马灯文字
'''
def main():
import os
import time
content = '北京欢迎你,为你开天辟地......'
while True:
# 清理出屏幕上输出
os.system('cls') # os.system('clear')
print(content)
# 休眠200毫秒
time.sleep(0.2)
content = content[1:] + content[0]
'''
# 练习2:设计一个函数产生制定长度的验证码,验证码由大小写字母和数字构成
'''
def generate_code(code_len=4):
"""
生成制定长度的验证码
:param code_len:验证码的长度(默认四个字符)
:return:由大小写因为字母和数字构成的随机验证码
"""
import random
all_chars = '0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ'
last_pos = len(all_chars) - 1
code = ''
for _ in range(code_len):
index = random.randint(0,last_pos)
code += all_chars[index]
return code
print(generate_code(10))
'''
# 练习3:设计一个函数返回给定文件名的后缀名
'''
def get_suffix(filename, has_dot=False):
"""
获取文件名的后缀
:param filename: 文件名
:param has_dot: 返回的后缀名是否需要带点
:return: 文件的后缀名
"""
pos = filename.rfind('.')
if 0 < pos < len(filename) -1:
index = pos if has_dot else pos + 1;
return filename[index:]
else:
return ''
print(get_suffix('简历.pdf',True))
'''
# 练习4:设计一个函数返回传入的列表中最大和第二大的元素
'''
def max2(x):
m1,m2 = (x[0], x[1]) if x[0] > x[1] else (x[1], x[0])
for index in range(2, len(x)):
if x[index] > m1:
m2 = m1
m1 = x[index]
elif x[index] > m2:
m2 = x[index]
return m1, m2
print(max2([1,2,3,4,5,6,7,8]))
'''
# 练习5:计算指定的年月日是这一年的第几天
'''
def is_leap_year(year):
"""
判断指定年是不是闰年
:param year: 年份
:return: 闰年返回True 平年返回False
"""
return year%4==0 and year%100!=0 or year%400==0
def which_day(year,month,date):
"""
计算传入的日期是这一年的第几天
:param year: 年
:param month: 月
:param date: 日
:return: 第几天
"""
days_of_month = [
[31,28,31,30,31,30,31,31,30,31,30,31],
[31,29,31,30,31,30,31,31,30,31,30,31]
][is_leap_year(year)]
total = 0
for index in range(month-1):
total += days_of_month[index]
return total + date
def main():
print(which_day(1980,11,29))
print(which_day(1981,12,31))
print(which_day(2018,1,1))
print(which_day(2018,3,1))
main()
'''
# 练习6:打印杨辉三角
'''
def main():
num = int(input('Number of rows:'))
yh = [[]] * num
for row in range(len(yh)):
yh[row] = [None] * (row + 1)
for col in range(len(yh[row])):
if col == 0 or col == row:
yh[row][col] = 1
else:
yh[row][col] = yh[row-1][col] + yh[row-1][col-1]
print(yh[row][col],end='\t')
print()
main()
'''
# 综合案例
# 案例1:双色球选号
'''
from random import randrange,randint,sample
# 使用random模块中的sample函数来实现从列表中选择不重复的N个元素
# enumerate() 函数用于将一个可遍历的数据对象(如列表、元组或字符串)组合为一个索引序列,
# 同时列出数据和数据下标,一般用在 for 循环当中。
def display(balls):
"""
输出列表中的双色球号码
"""
for index,ball in enumerate(balls):
if index == len(balls) - 1:
print('|',end=' ')
print('%-2d' % ball, end=' ')
print()
def random_select():
"""
随机选择一组号码
"""
red_balls = [x for x in range(1, 34)]
selected_balls = []
selected_balls = sample(red_balls, 6)
selected_balls.sort()
selected_balls.append(randint(1, 16))
return selected_balls
def main():
n = int(input('机选几注: '))
for _ in range(n):
display(random_select())
main()
'''
# 案例二:约瑟夫环问题
"""
《幸运的基督徒》
有15个基督徒和15个非基督徒在海上遇险,为了能让一部分人或下来,不得不将其中15个人扔到
海里面去,有个人想了个办法就是大家围城一圈,由某个人从1报数,报到9的人就扔到海里面,
他后面的人接着从一开始报数,报到9的人继续扔到海里面,直到扔掉15个人。
由于上帝的保佑,15个基督徒都幸免于难,问这些人最开始是怎末站的,那些位置是基督徒,
那些位置是非基督徒。
"""
'''
def main():
persons = [True] * 30
counter, index, number = 0, 0, 0
while counter < 15:
if persons[index]:
number += 1
if number == 9:
persons[index] = False
counter += 1
number = 0
index += 1
index %= 30
tmp = 0
for person in persons:
tmp += 1
print(('%d->基' if person else '%d->非')% tmp,end=' ')
main()
'''
# 案例三:井字棋游戏
'''
import os
def print_board(board):
print(board['TL'] + '|' + board['TM'] + '|' + board['TR'])
print('-+-+-')
print(board['ML'] + '|' + board['MM'] + '|' + board['MR'])
print('-+-+-')
print(board['BL'] + '|' + board['BM'] + '|' + board['BR'])
def main():
init_board = {
'TL':' ','TM':' ','TR':' ',
'ML':' ','MM':' ','MR':' ',
'BL':' ','BM':' ','BR':' '
}
begin = True
while begin:
curr_board = init_board.copy()
begin = False
turn = 'x'
counter = 0
os.system('clear')
print_board(curr_board)
while counter < 9:
move = input('轮到%s走棋,请输入位置:' % turn)
if curr_board[move] == ' ':
counter += 1
curr_board[move] = turn
if turn == 'x':
turn = 'o'
else:
turn = 'x'
os.system('clear')
print_board(curr_board)
choice = input('在玩一局?(yes|no)')
begin = choice == 'yes'
main()
'''
<file_sep># 总结和练习
# 1、求解100-999之间的所有“水仙花数”。
# 水仙花数是各位立方和等于这个数本身 153 = 1**3 + 5**3 + 3**3
'''
for num in range(100, 1000):
low = num % 10
mid = num // 10 % 10
high = num // 100
if num == low ** 3 + mid ** 3 + high ** 3:
print(num)
'''
# 2、找出1-9999之间的所有“完美数”。
# 完美数是除自身外其他所有因子的和正好等于这个数本身的数 6=1+2+3 28=1+2+4+7+14
'''
import time
import math
start = time.clock()
for num in range(1,10000):
sum = 0
for factor in range(1, int(math.sqrt(num)) + 1):
if num % factor == 0:
sum += factor
if factor > 1 and num / factor != factor:
sum += num / factor
if sum == num:
print(num)
end = time.clock()
print("执行时间:",(end - start),"秒")
'''
# 3、“百钱百鸡”问题。
# 1只公鸡5元 1只母鸡3元 3只小鸡1元 用100元买100只鸡 问公鸡 母鸡 小鸡各有多少只
'''
for x in range(0,20):
for y in range(0,33):
z = 100 - x - y
if 5*3 + 3*y +z/3 == 100:
print('公鸡:%d只, 母鸡:%d只, 小鸡:%d只' % (x, y, z))
'''
# 4、生成“斐波拉切数列”。
'''
a = 0
b = 1
count = 0;
for _ in range(50):
(a,b) = (b, a + b)
count += 1
'''
# 5、Craps赌博游戏。
'''
玩家摇两颗筛子 如果第一次摇出7点或11点 玩家胜
如果摇出2点 3点 12点 庄家胜 玩家输
若和为其他点数,则记录第一次的点数和,玩家继续摇筛子,直至点数和等于第一次摇出的点数和则玩家胜
若摇出的点数和为7,则抓庄家胜
玩家进入游戏时有1000元的赌注 全部输光游戏结束
'''
'''
from random import randint
money = 1000
while money > 0:
print('你的总资产为:', money)
needs_go_on = False
while True:
debt = int(input('请下注:'))
if debt > 0 and debt <= money:
break
first = randint(1, 6) + randint(1,6)
print('玩家摇出了%d点' % first)
if first == 7 or first == 1:
print('玩家胜!')
money += debt
elif first == 2 or first == 3 or first == 12:
print('庄家胜!')
money -= debt
else:
needs_go_on = True
while needs_go_on:
current = randint(1,6) + randint(1,6)
print('玩家摇出了%d点' % current)
if current == 7:
print('庄家胜!')
money -= debt
needs_go_on = False
elif current == first:
print('玩家胜!')
money += debt
needs_go_on = False
print('你破产了,游戏结束!')
'''
<file_sep>import socket
import struct
import json
# 1 买手机
phone = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
# 2 拨号
phone.connect(('127.0.0.1', 9094))
# 3 发、收消息
while True:
# 1 发命令
cmd = input('>> ').strip() # ls
if not cmd:
continue
phone.send(cmd.encode('utf-8'))
# 2 拿到命令的结果, 并打印
# 第一步:先收报头的长度
obj = phone.recv(4)
header_size = struct.unpack('i', obj)[0]
# 第二步: 在收报头
header_bytes = phone.recv(header_size)
# 第三步:从报头中解析出对真实数据的描述信息
header_json = header_bytes.decode('utf-8')
header_dict = json.loads(header_json)
print(header_dict)
total_size = header_dict['total_size']
# 第四步:接收真实的数据
recv_size = 0
recv_data=b''
while recv_size < total_size:
res= phone.recv(1024) # 1024是一个坑
recv_data += res
recv_size += len(res)
print(recv_data.decode('gbk'))
# 4 关闭
phone.close()
<file_sep>import socket
import subprocess
import struct
import json
import os
# 1 买手机
phone = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
phone.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1)
# 2 插手机卡
phone.bind(('127.0.0.1', 9094)) # bind传入的是一个元祖
# 3 开机
phone.listen(5) # 代表最大挂起的链接数
# 4 等电话
print("start....")
while True: # 链接循环
conn, client_addr = phone.accept()
print(client_addr)
# 5 收发消息
while True: # 通信循环
# 客户端单方面断开链接,会导致服务端死循环
try:
# 1 接收命令
res = conn.recv(8096) # b 'get a.txt'
if not res: # 适用于Linux系统
break
# 2 解析命令, 提取响应的参数
cmds = res.decode('utf-8').split() # ['get', 'a.txt']
filename = cmds[1]
# 3 以读的方式打开文件, 读取文件内容, 发送给客户端
# 第一步: 制作固定长度的报头
header_dict = {
'filename' : r'C:\Users\爱心天使\Desktop\1606990532.jpg',
'md5' : 'xxxfxx',
'filesize' : os.path.getsize(filename)
}
header_json = json.dumps(header_dict)
header_bytes = header_json.encode('utf-8')
# 第二步: 先发送报头的长度
conn.send(struct.pack('i', len(header_bytes)))
# 第三步:再发送报头
conn.send(header_bytes)
# 第四步:在发真实的数据
with open(filename, 'rb') as f:
# conn.send(f.read())
for line in f:
conn.send(line)
except ConnectionResetError: #使用于windows系统
break
conn.close()
phone.close()
<file_sep># 图形用户界面和游戏开发
"""
基于tkinter模块的GUI
GUI是图形用户界面的缩写,图形化的用户界面对使用过计算机的人来说应该都不陌生,在此无须进行
赘述.python默认的GUI开发模块是tkinter(在Python 3以前的版本中名为Tkinter),
从这个名字就可以看出它是基于Tk的,Tk是一个工具包,最初是为Tcl设计的,后来被移植到很多
其他的脚本语言中,它提供了跨平台的GUI控件。当然Tk并不是最新和最好的选择,
也没有功能特别强大的GUI控件,事实上,开发GUI应用并不是Python最擅长的工作,
如果真的需要使用Python开发GUI应用,wxPython、PyQt、PyGTK等模块都是不错的选择。
基本上使用tkinter来开发GUI应用需要以下五个步骤:
1、导入tkinter模块
2、创建一个顶层窗口对象并用它来承载整个GUI应用
3、在顶层窗口对象上添加GUI组件
4、通过代码将这些GUI组件的功能组织起来
5、进入主事件循环(main loop)
"""
'''
import tkinter
import tkinter.messagebox
def main():
flag = True
# 修改标签上的文字
def change_label_text():
nonlocal flag
flag = not flag
color,msg = ('red','hello world!') if flag else ('blue','goodbye world!')
label.config(text=msg,fg=color)
# 确认退出
def confirm_to_quit():
if tkinter.messagebox.askokcancel('温馨提示','确定要退出吗?'):
top.quit()
# 创建顶层窗口
top = tkinter.Tk()
# 设置窗口大小
top.geometry('240x160')
# 设置窗口标题
top.title('小游戏')
# 创建标签对象并添加到顶层窗口
label = tkinter.Label(top,text='hello world!',font='Arial -32',fg='red')
label.pack(expand=1)
# 创建一个装按钮的容器
panel = tkinter.Frame(top)
# 创建按钮对象 指定添加到那个容器 通过command参数绑定事件回调函数
button1 = tkinter.Button(panel,text='修改',command=change_label_text)
button1.pack(side='left')
button2 = tkinter.Button(panel,text='退出',command=confirm_to_quit)
button2.pack(side='right')
panel.pack(side='bottom')
tkinter.mainloop()
main()
'''
"""
需要说明的是,GUI应用通常是事件驱动式的,之所以要进入事件循环就是要监听鼠标,键盘等
各种事件的发生并执行相应的代码事件处理,因为事件会持续发生,所以需要这样的一个循环一直
运行着等待下一个事件的发生。另一方面,Tk为控件的摆放提供了三种布局管理器,通过布局管理器
可以对控件进行定位,这三种布局管理器分别是:Placer(开发者提供控件的大小和摆放位置),
Packer(自动将控件填充到合适的位置) 和 Grid(基于网络坐标来摆放控件)
"""
# 使用pygame进行游戏开发
"""
pygame是一个开源的python模块,专门用于多媒体应用(如电子游戏)的开发,其中包括对图像、
声音、视频、事件、碰撞等的支持。pygame建立在SDL的基础上,SDL是一套跨平台的多媒体开发库
用C语言实现,被广泛的应用于游戏、模拟器、播放器等的开发。而pygame让游戏开发者不再被底层
语言束缚,可以更多的关注游戏的功能和逻辑。
下面我们来完成一个简单的小游戏,游戏的名字叫“大球吃小球”,当然完成这个游戏并不是重点,
学会使用Pygame也不是重点,最重要的我们要在这个过程中体会如何使用前面讲解的
面向对象程序设计,学会用这种编程思想去解决现实中的问题。
"""
# 制作游戏窗口
'''
import pygame
def main():
# 初始化导入的pygame模块
pygame.init()
# 初始化用于显示的窗口并设置窗口尺寸
screen = pygame.display.set_mode((800,600))
# 设置当前窗口的标题
pygame.display.set_caption('大球吃小球')
running = True
# 开启一个事件循环处理发生的事件
while running:
# 从消息队列中获取事件并对事件进行处理
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
'''
# 在窗口中绘图
"""
可以使用pygame中的draw模块的函数在窗口上进行绘图,可以绘制的图形包括:线条、矩形、多边形
园、椭圆、圆弧等。需要说明的是,屏幕坐标系是将屏幕左上角设置为坐标原点(0,0),向右x轴的
正向,向下是y轴的正向,在表示位置或者设置尺寸时,我们默认的单位都是像素。所谓像素就是屏幕
上的一个点,你可以用浏览器的软件将一张图片放大若干倍,就可以看到这些点。pygame中表示颜色
用的是色光三原色表示法,即通过一个元祖或列表来表示颜色的RGB值,每个值都在0-255之间,因为
是每种原色都用一个8位(bit)的值来表示,三种颜色相当于一共24位构成,这也就是常说的"24位
颜色表示法"
"""
'''
import pygame
def main():
# 初始化导入的pygame模块
pygame.init()
# 初始化用于显示的窗口并设置窗口尺寸
screen = pygame.display.set_mode((800,600))
# 设置当前窗口的标题
pygame.display.set_caption('大球吃小球')
# 设置窗口的背景色(颜色是由红绿蓝三原色构成的元祖)
screen.fill((242,242,242))
# 绘制一个圆(参数分别是:屏幕,颜色,圆心位置,半径,0表示填充圆)
pygame.draw.circle(screen,(250,0,0),(100,100),30,0)
# 刷新当前窗口(渲染窗口将绘制的图象展示出来)
pygame.display.flip()
running = True
# 开启一个事件循环处理发生的事件
while running:
# 从消息队列中获取事件并对事件进行处理
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
main()
'''
# 加载图象
"""
如果需要直接加载图象到窗口上,可以使用pygame中image模块的函数来加载图象,在通过之前的
窗口对象的 blie 方法渲染图象
"""
'''
import pygame
def main():
# 初始化导入的pygame模块
pygame.init()
# 初始化用于显示的窗口并设置窗口尺寸
screen = pygame.display.set_mode((800,600))
# 设置当前窗口的标题
pygame.display.set_caption('大球吃小球')
# 设置窗口的背景色(颜色是由红绿蓝三原色构成的元祖)
screen.fill((255,255,255))
# 通过指定的文件名加载图象
ball_image = pygame.image.load('./timg.jpg')
# 在窗口上渲染图象
screen.blit(ball_image,(50,50))
# 刷新当前窗口(渲染窗口将绘制的图象展示出来)
pygame.display.flip()
running = True
# 开启一个事件循环处理发生的事件
while running:
# 从消息队列中获取事件并对事件进行处理
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
main()
'''
# 实现动画效果
"""
说到动画这个词大家都不会陌生事实上要实现动画效果,本身的原理也非常简单,就是将不连续的
图片连续的播放,只要每秒达到了一定的帧数,那么就可以做出比较流畅的动画效果。如果要让上面
代码中的小球动起来,可以将小球的位置用变量来表示,并在循环中修改小球的位置在刷新整个窗口
"""
'''
import pygame
def main():
# 初始化导入的pygame模块
pygame.init()
# 初始化用于显示的窗口并设置窗口尺寸
screen = pygame.display.set_mode((800,600))
# 设置当前窗口的标题
pygame.display.set_caption('大球吃小球')
# 定义变量来表示小球在屏幕上的位置
x,y = 50,50
running = True
# 开启一个事件循环处理发生的事件
while running:
# 从消息队列中获取事件并对事件进行处理
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
screen.fill((255,255,255)) # 设置窗口背景色
# 绘制一个圆(参数分别是:屏幕,颜色,圆心位置,半径,0表示填充圆)
pygame.draw.circle(screen,(250,0,0),(x,y),30,0)
pygame.display.flip() # 刷新当前窗口(渲染窗口将绘制的图象展示出来)
# 每隔50毫秒就改变小球的位置在刷新窗口
pygame.time.delay(50)
x,y = x + 5,y + 5
main()
'''
# 碰撞检测
"""
通常一个游戏中会有很多对对象出现,而这些对象之间的"碰撞"在所难免,比如炮弹击中飞机,箱子
撞到了地面等。碰撞检测在绝大多数的游戏中都是一个必须处理的至关重要的问题,pygame的sprite
(动画精灵)模块就提供了对碰撞检测的支持,这里不做介绍,因为要检测两个小球有没有碰撞只需要
检查球心的距离有没有小于两个球的半径之和。为了制造出更多的小球,我们可以通过对鼠标事件的
处理,在点击鼠标的位置创建颜色,大小和移动速度都随机的小球,当然要做到这一点需要把之前的
面向对象的知识应用起来
"""
'''
from enum import Enum
from math import sqrt
from random import randint
import pygame
# @uinque
class Color(Enum):
"""颜色"""
RED = (250,0,0)
GREEN = (0,250,0)
BLUE = (0,0,250)
BLACK = (0,0,0)
WHITE = (255,255,255)
GRAY = (242,242,242)
@staticmethod
def random_color():
"""获得随机颜色"""
r = randint(0,255)
g = randint(0,255)
b = randint(0,255)
return (r,g,b)
class Ball(object):
"""球"""
def __init__(self,x,y,radius,sx,sy,color=Color.RED):
"""初始化方法"""
self.x = x
self.y = y
self.radius = radius # 球的半径
self.sx = sx
self.sy = sy
self.color = color
self.alive = True
def move(self,screen):
"""移动"""
self.x += self.sx
self.y += self.sy
if self.x - self.radius <= 0 or self.x - self.radius >= screen.get_width():
self.sx = -self.sx
if self.y - self.radius <= 0 or self.y + self.radius >= screen.get_height():
self.sy = -self.sy
def eat(self,other):
"""吃其他球"""
if self.alive and other.alive and self != other:
dx,dy = self.x - other.x,self.y - other.y
distance = sqrt(dx**2 + dy**2)
if distance < self.radius + other.radius and self.radius > other.radius:
other.alive = False
self.radius = self.radius + int(other.radius*0.146)
def draw(self,screen):
"""在窗口上绘制球"""
pygame.draw.circle(screen,self.color,(self.x,self.y),self.radius,0)
# 事件处理
"""
可以在事件循环中对鼠标事件进行处理,通过事件对象的 type 属性可以判定事件类型,在通过
pos 属性就可以获得鼠标点击的位置。如果要处理键盘事件也是在这个地方,做法和处理鼠标事件
类似
"""
def main():
# 定义用来装所有球的容器
balls = []
# 初始化导入的pygam模块
pygame.init()
# 初始化用于显示的窗口并设置窗口尺寸
screen = pygame.display.set_mode((800,600))
# 设置当前窗口的标题
pygame.display.set_caption('大球吃小球')
running = True
# 开启一个事件循环处理发生的事件
while running:
# 从消息队列中获取事件并对事件进行处理
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 处理鼠标事件的代码
if event.type == pygame.MOUSEBUTTONDOWN and event.button == 1:
# 获得点击鼠标的位置
x,y = event.pos
radius = randint(10,100)
sx,sy = randint(-10,10),randint(-10,10)
color = Color.random_color()
# 在点击鼠标的位置创建一个球(大小,速度和颜色随机)
ball = Ball(x,y,radius,sx,sy,color)
# 将球添加到列表容器中
balls.append(ball)
screen.fill((255,255,255))
# 取出容器中的球 如果没被吃掉就绘制 被吃掉了就移除
for ball in balls:
if ball.alive:
ball.draw(screen)
else:
balls.remove(ball)
pygame.display.flip() # 刷新当前窗口(渲染窗口将绘制的图象展示出来)
# 每隔50毫秒就改变球的位置在刷新窗口
pygame.time.delay(50)
for ball in balls:
ball.move(screen)
# 检查有没有吃到其他的球
for other in balls:
ball.eat(other)
main()
'''
<file_sep>import socket
import struct
import json
# 1 买手机
phone = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
# 2 拨号
phone.connect(('127.0.0.1', 9094))
# 3 发、收消息
while True:
# 1 发命令
cmd = input('>> ').strip() # get a.txt
if not cmd:
continue
phone.send(cmd.encode('utf-8'))
# 2 接收文件的内容,以写的方式打开一个新文件,接收服务端发来的文件内容,写入客户端的新文件
# 第一步:先收报头的长度
obj = phone.recv(4)
header_size = struct.unpack('i', obj)[0]
# 第二步: 在收报头
header_bytes = phone.recv(header_size)
# 第三步:从报头中解析出对真实数据的描述信息
header_json = header_bytes.decode('utf-8')
header_dict = json.loads(header_json)
print(header_dict)
total_size = header_dict['filesize']
filename = header_dict['filename']
# 第四步:接收真实的数据
with open(filename, 'wb') as f:
recv_size = 0
while recv_size < total_size:
line= phone.recv(1024) # 1024是一个坑
f.write(line)
recv_size += len(line)
# 4 关闭
phone.close()
<file_sep>import socket
import time
# 1 买手机
server = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1)
# 2 插手机卡
server.bind(('127.0.0.1', 9094)) # bind传入的是一个元祖
# 3 开机
server.listen(5) # 代表最大挂起的链接数
conn, addr = server.accept()
res1 = conn.recv(1)
print('第一次', res1)
time.sleep(6)
res2 = conn.recv(1024)
print('第二次', res2)
<file_sep>import socket
# 1 买手机
phone = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
phone.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1)
# 2 插手机卡
phone.bind(('127.0.0.1', 9094)) # bind传入的是一个元祖
# 3 开机
phone.listen(5) # 代表最大挂起的链接数
# 4 等电话
print("start....")
conn, client_addr = phone.accept()
print(client_addr)
# 5 收发消息
while True: # 通信循环
# 客户端单方面断开链接,会导致服务端死循环
try:
data = conn.recv(1024) # 1 单位: bytes 2 1024代表最大接收1024个字节
if not data: # 适用于Linux系统
break
print('客户端数据', data)
conn.send(data.upper
except ConnectionResetError: # 使用于windows系统
break
conn.close()
phone.close()
<file_sep>import struct
import json
header_dict = {
'filename' : 'a.txt',
'md5' : 'xxxfxx',
'total_size' : 33333333333333333333333333333333333333
}
header_json = json.dumps(header_dict)
print(header_json, type(header_json))
header_bytes = header_json.encode('utf-8')
# print(header_bytes)
print(len(header_bytes))
struct.pack('i', len(header_bytes))
<file_sep># 文件和异常
"""
在实际开发中,常常需要对程序中的数据进行持久化操作,而实现数据持久化最简单的方式就是将
数据保存到文件中。说到"文件"这个词,需要先了解一下文件系统,这个自行了解
在python中实现文件的读写操作非常简单,通过python内置的open函数,我们可以指定文件名,
操作模式,编码信息等来获得操作文件的对象,接下来就可以对文件进行读写操作了。这里的操作
模式指要打开的文件(字符文件还是二进制文件)以及做什么样的操作(读,写还是追加)。
操作模式 具体含义
'r' 读取 (默认)
'w' 写入(会先截断之前的内容)
'x' 写入,如果文件已经存在会产生异常
'a' 追加,将内容写入到已有文件的末尾
'b' 二进制模式
't' 文本模式(默认)
'+' 更新(既可以读又可以写)
"""
# 读文本文件
"""
读取文本文件时,需要在使用open函数时指定好带路劲的文件名(可以使用相对路径或绝对路径)
并将文件模式设置为"r"(如果不指定,默认值是"r"),然后通过 encoding 参数指定编码(如果
不指定,默认是None,那么在读取文件时使用的是操作系统默认的编码),如果不能保证文件使用的
编码方式与encoding参数指定的编码方式一致,那么就可能无法解码字符而导致读取失败。
下面的例子演示如何读取一个纯文本文件
"""
'''
def main():
f = open('致橡树.txt', 'r', encoding='utf-8')
print(f.read())
f.close()
main()
'''
""""
请注意上面的代码,如果open函数指定的文件并不存在或者无法打开,那么将引发异常状况导致
程序崩溃。为了让代码有一定的健壮性和容错性,我们可以使用Python的异常机制
对可能在运行时发生状况的代码进行适当的处理,如下所示。
"""
'''
def main():
f = None
try:
f= open('致橡树.txt', 'r', encoding='utf-8')
print(f.read())
except FileNotFoundError:
print('无法打开指定文件')
except LookupError:
print('指定了位置的编码')
except UnicodeDecodeError:
print('读取文件时解码错误')
finally:
if f:
f.close()
main()
'''
"""
在Python中,我们可以将那些在运行时可能会出现状况的代码放在try代码块中,在try代码块的后面可以跟上
个或多个except来捕获可能出现的异常状况。例如在上面读取文件的过程中,文件找不到会引发
FileNotFoundError,指定了未知的编码会引发LookupError,而如果读取文件时无法按指定方式解码会引发
UnicodeDecodeError,我们在try后面跟上了三个except分别处理这三种不同的异常状况。
最后我们使用finally代码块来关闭打开的文件,释放掉程序中获取的外部资源,由于finally块的代码不论程序正常还是异
常都会执行到(甚至是调用了sys模块的exit函数退出Python环境,finally块都会被执行,因为exit函数
实质上是引发了SystemExit异常),因此我们通常把finally块称为“总是执行代码块”,它最适合用来做释放外
部资源的操作。如果不愿意在finally代码块中关闭文件对象释放资源,也可以使用上下文语法,通过with关键
字指定文件对象的上下文环境并在离开上下文环境时自动释放文件资源,代码如下所示
"""
'''
def main():
try:
with open('致橡树.txt','r', encoding='utf-8') as f:
print(f.read())
except FileNotFoundError:
print('无法打开指定文件')
except LookupError:
print('指定了未知的编码')
except UnicodeDecodeError:
print('读取文件时解码错误')
main()
'''
"""
除了使用文件对象的read方法读取文件之外,还可以使用for-in循环逐行读取或者使用readlines方法
将文件按行读取到一个列表容器中。
"""
'''
import time
def main():
# 一次性读取整个文件内容
with open('致橡树.txt','r',encoding='utf-8') as f:
print(f.read())
# 通过for-in循环逐行读取
with open('致橡树.txt',mode='r',encoding='utf-8') as f:
for line in f:
print(line,end='')
time.sleep(0.5)
print()
# 读取文件按行读取到列表中
with open('致橡树.txt',encoding='utf-8') as f:
lines = f.readlines()
print(lines)
main()
'''
"""
要将文本信息写入文件也非常简单,在使用open函数时指定好文件名字并将文件模式设置为'w',
注意需要对文件内容进行追加写入,应该讲模式设置为'a'。如果要写入的文件不存在会自动创建
文件而不是引发一场。
下面的例子演示了如何将1-9999之间的素数分别写入三个文件中(1-99之间的素数保存在a.txt中,
100-999之间的素数保存在b.txt中,1000-9999之间的素数保存在c.txt中)。
"""
'''
from math import sqrt
def is_prime(n):
"""判断素数的函数"""
assert n > 0
for factor in range(2,int(sqrt(n))+1):
if n % factor == 0:
return False
return True if n != 1 else False
def main():
filenames = ('a.txt','b.txt','c.txt')
fs_list = []
try:
for filename in filenames:
fs_list.append(open(filename,'w',encoding='utf-8'))
for number in range(1,10000):
if is_prime(number):
if number < 100:
fs_list[0].write(str(number)+'\n')
elif number < 1000:
fs_list[1].write(str(number)+'\n')
else:
fs_list[2].write(str(number)+'\n')
except IOError as ex:
print(ex)
print('写文件时发生错误')
finally:
for fs in fs_list:
fs.close()
print('操作完成')
main()
'''
# 读写二进制文件
"""知道了如何读写文本文件要读写二进制文件也就很简单了,下面的代码实现了复制图片文件的功能"""
'''
def main():
try:
with open('timg.jpg','rb') as fs1:
data = fs1.read() # <class 'bytes'>
print(type(data))
with open('皮卡丘jpg','wb') as fs2:
fs2.write(data)
except FileNotFoundError as e:
print('指定的文件无法打开')
except IOError as e:
print('读写文件时出现错误')
print('程序结束')
main()
'''
# 读取json文件
"""
如果希望吧一个列表或者一个字典中的数据保存到文件中该怎么做呢?答案是将数据以json格式保存,
JSON是“JavaScript Object Notation”的缩写,它本来是JavaScript语言中创建对象的一种字面量语法,
现在已经被广泛的应用于跨平台跨语言的数据交换,原因很简单,因为JSON也是纯文本,任何系统任何编程语
言处理纯文本都是没有问题的。目前JSON基本上已经取代了XML作为异构系统间交换数据的事实标准。
关于JSON的知识,更多的可以参考JSON的官方网站,从这个网站也可以了解到每种语言处理JSON数据格式
可以使用的工具或三方库,下面是一个JSON的简单例子。
"""
'''
{
"name":"爱心天使",
"age":23,
"qq":123456,
"friends":["蔡文姬","曹操"],
"cars":[
{"brand":"BYD","max_speed":1800},
{"brand":"Audi","max_speed":280},
{"brand":"Benz","max_speed":320}
]
}
可能大家已经注意到了,上面的JSON跟Python中的字典其实是一样一样的,
事实上JSON的数据类型和Python的数据类型是很容易找到对应关系的,如下面两张表所示。
'''
"""
JSON Python
---------------------------------------------------------
object dict
array list
string str
number (int / real) int / float
true / false True / False
null None
++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
Python JSON
----------------------------------------------------------
dict object
list, tuple array
str string
int, float, int- & float-derived Enums number
True / False true / false
None null
++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
"""
"""我们可以使用Python中的json模块就可以将字典或列表以json格式保存到文件中"""
'''
import json
def main():
mydict = {
"name":"爱心天使",
"age":23,
"qq":123456,
"friends":["蔡文姬","曹操"],
"cars":[
{"brand":"BYD","max_speed":1800},
{"brand":"Audi","max_speed":280},
{"brand":"Benz","max_speed":320}
]
}
try:
with open('data.json','w',encoding='utf-8') as fs:
json.dump(mydict,f)
except IOError as e:
print(e)
print("数据保存完成")
main()
'''
"""
json 模块有四个比较重要的函数,分别是:
dump-将python对象按照json格式序列化到文件中
dumps-将python对象处理成json格式的字符串
load-将文件中的json数据反序列化成对象
loads-将字符串的内容反序列化成python对象
"""
"""
这里出现了两个概念,一个叫序列化,一个叫反序列化。自由的百科全书维基百科上对这两个概念是这样解
释的:“序列化(serialization)在计算机科学的数据处理中,是指将数据结构或对象状态转换为可以存储或
传输的形式,这样在需要的时候能够恢复到原先的状态,而且通过序列化的数据重新获取字节时,可以利用
这些字节来产生原始对象的副本(拷贝)。与这个过程相反的动作,即从一系列字节中提取数据结构的操
作,就是反序列化(deserialization)”。
目前绝大多数网络数据服务(或称之为网络API)都是基于HTTP协议提供JSON格式的数据,关于HTTP协议
的相关知识,可以看看阮一峰老师的《HTTP协议入门》,如果想了解国内的网络数据服务,可以看看聚合
数据和阿凡达数据等网站,国外的可以看看{API}Search网站。下面的例子演示了如何使用requests模块(封
三方网络访问模块)访问网络API获取国内新闻,如何通过json模块解析JSON数据并显示新闻标题,这个例子使
用了天行数据提供的国内新闻数据接口,其中的APIKey需要自己到该网站申请
"""
'''
——————————————————————————————————————————————————————————————————————————————————————————————————————
import requests
import json
def main():
resp = requests.get('http://api.tianapi.com/guonei/?key=<KEY>&num=11')
data_model = json.loads(resp.text)
for news in data_model['newslist']:
print(news['title'])
main()
'''
'''
另外,如果要了解更多的关于Python异常机制的知识,可以看看segmentfault上面的文章《总结:Python中的异常处理》
,这篇文章不仅介绍了Python中异常机制的使用,还总结了一系列的最佳实践,很值得一读。
链接:https://segmentfault.com/a/1190000007736783
'''
<file_sep>import sys
import pygame
from settings import Settings
from ship import Ship
import game_functions as gf
from pygame.sprite import Group
from alien import Alien
from game_stats import GameStats
from button import Button
def run_game():
#初始化游戏并创建一个屏幕对象
pygame.init()
ai_settings = Settings()
screen = pygame.display.set_mode((ai_settings.screen_width, ai_settings.screen_height))
pygame.display.set_caption("Alien Invansion")
#创建一个用于存储游戏统计信息的实例
stats = GameStats(ai_settings)
#创建一艘飞船,一个子弹编组和一个外星人编组
ship = Ship(ai_settings, screen)
bullets = Group()
aliens = Group()
#创建外星人群
gf.creat_fleet(ai_settings, screen, ship, aliens)
#创建play按钮
#play_button = Button(ai_settings, screen, "Play")
#开始游戏主循环
while True:
gf.check_events(ai_settings, screen, ship,bullets)
if stats.game_active:
ship.update()
gf.update_bullets(ai_settings, screen,ship, aliens, bullets)
gf.update_aliens(ai_settings,stats,screen, ship, aliens, bullets)
gf.update_screen(ai_settings, screen,stats, ship,aliens, bullets)
run_game()
| 7e84fe43a0728e2e10b4ce4e50ad93b0c8a8ac9e | [
"Python"
] | 33 | Python | heartangle/python | 712f9a946797de6e6e712f5bc4ba872fe0e6d572 | 418c203a7384de53ff4bd95e85fc7286937df953 | |
refs/heads/master | <repo_name>Varunastra/SpringTest<file_sep>/js/main.js
const projectsData = [
{
title: "Spring boot",
description: `Takes an opinionated view of building Spring applications and gets
you up and running as quickly as possible.`,
imageClass: "boot-logo"
},
{
title: "Spring framework",
description: `Provides core support for dependency injection, transaction
management, web apps, data access, messaging and more.`,
imageClass: "leaf-logo"
},
{
title: "Spring cloud data flow",
description: `An orchestration service for composable data microservice
applications on modern runtimes.`,
imageClass: "flow-logo"
},
{
title: "Spring cloud",
description: `Provides a set of tools for common patterns in distributed
systems. Useful for building and deploying microservices.`,
imageClass: "spring-logo"
},
{
title: "Spring data",
description: `Provides a consistent approach to data access – relational,
non-relational, map-reduce, and beyond.`,
imageClass: "data-logo"
},
{
title: "Spring integration",
description: `Supports the well-known
<em>Enterprise Integration Patterns</em> via lightweight messaging
and declarative adapters.`,
imageClass: "integration-logo"
}
];
function serializeProject(project) {
return `<div class="project">
<div class="project__img__${project.imageClass}"></div>
<h2 class="project__title">${project.title}</h2>
<div class="project__description">
${project.description}
</div>
</div>`;
}
function serializeProjects(projects) {
return projects.map(serializeProject);
}
function loadProjects(data = null) {
const projects = document.querySelector(".projects");
data
? (projects.innerHTML = data)
: (projects.innerHTML = serializeProjects(projectsData));
}
function setDesktopEvents() {
const searchBtn = document.querySelector("#searchBtn-desktop");
searchBtn.addEventListener("click", () => {
const searchBar = document.querySelector(".searchbar-desktop");
const searchImg = searchBtn.childNodes[1];
if (!searchBar.className.includes("show")) {
searchBar.classList.add("searchbar-show");
searchImg.className = searchImg.className.replace(
"search-btn",
"close-btn"
);
searchBtn.className = searchBtn.className.replace(
"--normal",
"--selected"
);
} else {
searchBar.className = "searchbar-desktop";
searchBtn.className = searchBtn.className.replace(
"--selected",
"--normal"
);
searchImg.className = searchImg.className.replace(
"close-btn",
"search-btn"
);
}
});
const searchBarBtn = document.querySelector(".searchbar-desktop__btn");
searchBarBtn.addEventListener("click", () => {
searchProjectsDesktop();
});
const desktopInput = document.getElementById("searchInput-desktop");
desktopInput.addEventListener("change", () => {
searchProjectsDesktop();
});
}
function setMobileEvents() {
const panelBtn = document.querySelector(".header-mobile__links");
const sidePanel = document.querySelector(".sidepane");
const headerMobile = document.querySelector(".header-mobile");
const navCapture = document.querySelector("#nav-capture");
panelBtn.addEventListener("click", () => {
sidePanel.classList.add("sidepane-transform");
headerMobile.classList.add("header-transform");
navCapture.classList.add("sidepane-capture");
document.body.style.overflowY = "hidden";
});
navCapture.addEventListener("click", () => {
sidePanel.classList.remove("sidepane-transform");
headerMobile.classList.remove("header-transform");
navCapture.classList.remove("sidepane-capture");
document.body.style.overflowY = "scroll";
});
const mobileInput = document.getElementById("searchInput-mobile");
mobileInput.addEventListener("change", () => {
searchProjectsMobile();
});
const searchBtnSidepane = document.querySelector(".sidepane__search-btn");
searchBtnSidepane.addEventListener("click", () => {
searchProjectsMobile();
});
}
function searchProjects(title) {
const desktopInput = document.getElementById("searchInput-desktop");
const mobileInput = document.getElementById("searchInput-mobile");
desktopInput.value = mobileInput.value = title;
const newData = projectsData.filter(elem => {
if (elem.title.toLowerCase().includes(title.toLowerCase())) return true;
});
if (newData.length) {
const serliazedData = serializeProjects(newData);
loadProjects(serliazedData);
} else {
document.querySelector(".projects").innerHTML = "<h1>No results</h1>";
}
}
function searchProjectsDesktop() {
const desktopInput = document.getElementById("searchInput-desktop").value;
searchProjects(desktopInput);
}
function searchProjectsMobile() {
const mobileInput = document.getElementById("searchInput-mobile").value;
searchProjects(mobileInput);
}
window.onload = () => {
setDesktopEvents();
setMobileEvents();
loadProjects();
};
| 52d8b4daf4663bb37a9a51bd617405dea6063041 | [
"JavaScript"
] | 1 | JavaScript | Varunastra/SpringTest | e5332070bbfa2c52605f8e1ba72d213903536d95 | 061f51fe0cb695ead167c47e113ff524ae9a4a50 | |
refs/heads/master | <repo_name>Nalox1/iotsystem<file_sep>/webservice/src/main/webapp/dashboardWebsocket.js
var wsRealtime;
var wsHistory;
var pathRealtime = "ws://" + location.hostname + (location.port ? ":" + location.port: "") + "/webservice/websocket/realtime/dashboard";
var pathHistory = "ws://" + location.hostname + (location.port ? ":" + location.port: "") + "/webservice/websocket/history";
function connectRealtime()
{
wsRealtime = new WebSocket(pathRealtime);
wsRealtime.onmessage = function(event)
{
var receivedObject = JSON.parse(event.data);
var measurementString = receivedObject.description;
measurementString += "<br>" + receivedObject.dateTime;
if (receivedObject.hasOwnProperty("temperature"))
{
measurementString += "<br>Temperature: " + receivedObject.temperature + " °C";
}
if (receivedObject.hasOwnProperty("humidity"))
{
measurementString += "<br>Humidity: " + receivedObject.humidity + " %";
}
if (receivedObject.hasOwnProperty("radiation"))
{
measurementString += "<br>Radiation: " + receivedObject.radiation + " µSv/h";
}
if (receivedObject.hasOwnProperty("light"))
{
measurementString += "<br>Light: " + receivedObject.light + " lux";
}
document.getElementById(receivedObject.mac).innerHTML = measurementString;
};
}
function connectHistory()
{
console.log("Connecting to history websocket...");
wsHistory = new WebSocket(pathHistory);
console.log("Connected to history websocket.");
wsHistory.onmessage = function(event)
{
document.getElementById("history").innerHTML = event.data;
console.log(event.data);
};
}<file_sep>/device/esp8266-01/config.h
#define DEVICE_ID WiFi.macAddress()
#define DEVICE_SEND_INTERVAL 1000
#define DHT_PIN 2
#define DHT_TYPE DHT11
#define MESSAGE_MAX_LEN 256
#define WEBSOCKET_PATH_LENGTH 256
WebSocketClient websocketClient;
WiFiClient wifiClient;
unsigned long previousMillis = 0;
// WiFi settings
const static char* WIFI_SSID = "WIFI_SSID";
const static char* WIFI_PASS = "<PASSWORD>";
// Websocket settings
String connectionString = "/webservice/websocket/realtime/" + DEVICE_ID;
char* webserverHost = "echo.websocket.org";
const int webserverPort = 8080;
char websocketPath[WEBSOCKET_PATH_LENGTH];
// DHT11 Sensor
static DHT dht(DHT_PIN, DHT_TYPE);
| 4c8aaf89a0d990e3f8a28bcaeb217888543b4964 | [
"JavaScript",
"C"
] | 2 | JavaScript | Nalox1/iotsystem | b169a7f73b47678a377e6d3b06f586c0acaba5c9 | aabd8ef6215a1651012b973f56a6d3fafe18bdd0 | |
refs/heads/master | <file_sep>package com.travelmanagement.repositories;
import org.springframework.data.repository.CrudRepository;
import com.travelmanagement.models.UserLogin;
public interface UserRepository extends CrudRepository<UserLogin,String>{
public UserLogin findByName(String name);
}
<file_sep># travel-history-management-system-springboot
<file_sep>package com.travelmanagement.models;
import java.util.Date;
public class Country {
private Date startdate;
private Date enddate;
private int diffInDays;
public Country() {
super();
}
public Country(Date startdate, Date enddate,int diffInDays) {
super();
this.startdate = startdate;
this.enddate = enddate;
this.diffInDays=diffInDays;
}
public Date getStartdate() {
return startdate;
}
public void setStartdate(Date startdate) {
this.startdate = startdate;
}
public Date getEnddate() {
return enddate;
}
public void setEnddate(Date enddate) {
this.enddate = enddate;
}
public int getDiffInDays() {
return diffInDays;
}
public void setDiffInDays(int diffInDays) {
this.diffInDays = diffInDays;
}
@Override
public String toString() {
return "Country [startdate=" + startdate + ", enddate=" + enddate + ", diffInDays=" + diffInDays + "]";
}
}
<file_sep>package com.travelmanagement.services;
import java.util.ArrayList;
import java.util.List;
import java.util.Optional;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.travelmanagement.models.Country;
import com.travelmanagement.models.Travel;
import com.travelmanagement.models.UserLogin;
import com.travelmanagement.repositories.TravelRepository;
import com.travelmanagement.repositories.UserRepository;
@Service
public class TravellerService {
@Autowired
TravelRepository travelrepository;
@Autowired
UserRepository userRepository;
public List<Country> getTravelsByCountry(String des,String name) {
List<Country> lst = new ArrayList<Country>();
/*
* travelrepository.findByDes(country).forEach(x->{ lst.add(new
* Country(x.getName(),x.getSt(),x.getEn())); });
*/
travelrepository.findByDesAndUserloginName(des, name).forEach(x->{
lst.add(new Country(x.getSt(),x.getEn(),(int)((x.getEn().getTime() - x.getSt().getTime()) / (1000 * 60 * 60 * 24))));
});
System.out.println(lst);
return lst;
}
public boolean getUserValidity(UserLogin user) {
if(userRepository.findByName(user.getName())==null)
return false;
String x=userRepository.findByName(user.getName()).getName();
String y=userRepository.findByName(user.getName()).getPass();
String z=user.getName();
String w=user.getPass();
if(x!=null&&x.equals(z)&&y!=null&&y.equals(w))
{
return true;
}
else
{
return false;
}
}
public String signup(UserLogin user) {
if(userRepository.findByName(user.getName())==null)
{
userRepository.save(user);
return "Signup successful!!";
}
else
{
return null;
}
}
public boolean addTravels(Travel travel) {
if(userRepository.findByName(travel.getUserlogin().getName())==null)
return false;
travelrepository.save(travel);
return true;
}
public void updateTravel(Travel travel,String name,int id) {
travel.setUserlogin(new UserLogin(name,""));
travel.setId(id);
travel.setName(travelrepository.findById(id).get().getName());
travel.setSrc(travelrepository.findById(id).get().getSrc());
travel.setSt(travelrepository.findById(id).get().getSt());
travelrepository.save(travel);
}
public Optional<Travel> getTravel(int id) {
return travelrepository.findById(id);
}
public List<Travel> getAllTravels(String name) {
List<Travel> lst=new ArrayList<>();
travelrepository.findByUserloginName(name).forEach(lst::add);
return lst;
}
}
| cf501a43603fda0b0a0f6e404b1a5729bbe27d9d | [
"Markdown",
"Java"
] | 4 | Java | kunalmet16/travel-history-management-system-springboot | 0d5e41459113d54368f0af0faa25c405f81f602a | e76a14d8ad4d611ada58c47749680e5029c1e61a | |
refs/heads/master | <file_sep>{
#include <cstdlib>
// #include <iostream>
#include <fstream>
#include <stdio.h>
#include <string.h>
#include <vector>
#include <math.h>
#include <TH1F.h>
using namespace std;
gROOT->Reset();
gROOT->SetStyle("Plain");
// DEFINING FILE AND TREE VARIABLES
const int NENERGIES= 1;
const int nATT = 10;
TFile * RunFile[NENERGIES];
RunFile[0] = new TFile("data/1GeV_test_Attenuation_curves.root","READ"); //
// RunFile[1] = new TFile("data/50GeV_full_sim_1mind.root","READ"); //
// RunFile[2] = new TFile("data/50GeV_full_sim_2mind.root","READ"); //
// RunFile[3] = new TFile("data/50GeV_full_sim_3mind.root","READ"); //
// RunFile[4] = new TFile("data/50GeV_full_sim_5mind.root","READ"); //
// RunFile[5] = new TFile("data/50GeV_full_sim_7mind.root","READ"); //
double input_energy[NENERGIES];
input_energy[0] = 1;
// input_energy[1] = 20;
// input_energy[2] = 50;
// input_energy[3] = 100;
// input_energy[4] = 120;
// input_energy[5] = 150;
double input_att[nATT];
input_att[0] = 0.117681;
input_att[1] = 0.0637243;
input_att[2] = 0.0439325;
input_att[3] = 0.0321204;
input_att[4] = 0.0192074;
input_att[5] = 0.0124216;
input_att[6] = 0.00660631;
input_att[7] = 0.00431397;
input_att[8] = 0.00229227;
input_att[9] = 0.000864042;
// input_att[10] = 0.001;
cout << "almeno fin qui ..." << endl;
TGraphErrors * grResolution = new TGraphErrors ();
TGraphErrors * grLinearity = new TGraphErrors ();
TGraphErrors * grResolutionAtt [nATT];
TGraphErrors * grLinearityAtt [nATT];
for (int iAtt = 0; iAtt < nATT; iAtt++) {
grResolutionAtt[iAtt] = new TGraphErrors ();
grLinearityAtt[iAtt] = new TGraphErrors ();
}
cout << "almeno fin qui ..." << endl;
TH1F * hDepoEn[NENERGIES];
TH1F * hAttEn[NENERGIES][nATT];
TH1F * hAttCalib_En[NENERGIES][nATT];
TH1F * hInputAbsorption[NENERGIES];
int NBINS = 1000;
char name[50];
for (int iEn = 0; iEn < NENERGIES; iEn ++) {
sprintf (name, "hDepoEn_%d", iEn);
hDepoEn [iEn] = new TH1F (name, name, NBINS, 0.3*input_energy[iEn], input_energy[iEn]);
for (int iAtt = 0; iAtt < nATT; iAtt++){
sprintf (name, "hAttEn_%d_ATT_%d", iEn, iAtt);
// hAttEn[iEn][iAtt] = new TH1F (name, name, NBINS, 0.3*input_energy[iEn]*input_att[iAtt], input_energy[iEn]*input_att[iAtt]);
hAttEn[iEn][iAtt] = new TH1F (name, name, NBINS, 0, input_energy[iEn]*input_att[iAtt]*2);
sprintf (name, "hAttCalib_En_%d_ATT_%d", iEn, iAtt);
hAttCalib_En[iEn][iAtt] = new TH1F (name, name, NBINS, 0.3*input_energy[iEn], input_energy[iEn]*2);
}
}
/// defining tree variables
Float_t InitialPositionX;
Float_t InitialPositionY;
Float_t InitialPositionZ;
Float_t Total_energy ;
Float_t Total_ion_energy ;
Float_t Total_ion_energy_att[nATT] ;
///******************************************///
/// Run over different beam position
///******************************************///
for (int iEn = 0; iEn < NENERGIES; iEn++) {
TTree* TreeRun = (TTree*) RunFile[iEn].Get("tree");
hInputAbsorption[iEn] = (TH1F*) RunFile[iEn].Get("hAttenuation");
TreeRun->SetBranchAddress("InitialPositionX",&InitialPositionX);
TreeRun->SetBranchAddress("InitialPositionY",&InitialPositionY);
TreeRun->SetBranchAddress("InitialPositionZ",&InitialPositionZ);
TreeRun->SetBranchAddress("Total_energy",&Total_energy);
TreeRun->SetBranchAddress("Total_ion_energy",&Total_ion_energy);
TreeRun->SetBranchAddress("Total_ion_energy_att",&Total_ion_energy_att);
///******************************************///
/// Run over events ///
///******************************************///
int NEVENTS = TreeRun->GetEntries();
cout << "iEn = " << iEn << " :: nEvents = " << NEVENTS << endl;
for (Int_t iEvt= 0; iEvt < NEVENTS; iEvt++) {//&& iEvt < 5
TreeRun->GetEntry(iEvt);
cout << " iEvt = " << iEvt << endl;
hDepoEn[iEn]->Fill(Total_ion_energy);
for (int iAtt = 0; iAtt < nATT; iAtt++){
cout << "input_att[" << iAtt << "] = " << Total_ion_energy_att[iAtt]/0.84 <<";" << endl;
hAttEn[iEn][iAtt]->Fill(Total_ion_energy_att[iAtt]);
}
} //end of events loop
/*
TF1 * fEnergies = new TF1 ("fEnergies", " [0]*exp((x-220)/[1]) + [2]", 0, 220);
fEnergies->SetParameters(0.1, 20, 0.01);
// fEnergies->FixParameter(1,220);
fEnergies->SetLineColor(iEn+1);
for (int j=0; j< 10; j++) hEfficiency[iEn]->Fit("fEnergies", "QR");
TF1 * fDecayTime = new TF1 ("fDecayTime", " [0]*exp(-x/[1])", 0, 100);
fDecayTime->SetParameters(100, 20);
fDecayTime->SetLineColor(iEn+1);
for (int j = 0; j< 10; j++) hPulseShape[iEn]->Fit("fDecayTime", "QR");
*/
hInputAbsorption[iEn]->SetLineColor(iEn+1);
hDepoEn[iEn]->SetLineColor(iEn+1);
for (int iAtt = 0; iAtt < nATT; iAtt++) hAttEn[iEn][iAtt]->SetLineColor(iEn+1);
// if (iEn == 0) double initialLY = hLightOutput[0]->GetMean();
grResolution->SetPoint(iEn, input_energy[iEn], hDepoEn[iEn]->GetRMS()/hDepoEn[iEn]->GetMean());
grLinearity->SetPoint(iEn, input_energy[iEn], hDepoEn[iEn]->GetMean()/input_energy[iEn]);
for (int iAtt = 0; iAtt < nATT; iAtt++){
grResolutionAtt[iAtt]->SetPoint(iEn, input_energy[iEn], hAttEn[iEn][iAtt]->GetRMS()/hAttEn[iEn][iAtt]->GetMean());
// grResolution[iAtt]->SetPointError(iEn, 0, hLightOutput[iEn]->GetMeanError());
grLinearityAtt[iAtt]->SetPoint(iEn, input_energy[iEn], hAttEn[iEn][iAtt]->GetMean()/input_energy[iEn]);
}
} //end of energy loop
/// ********* DRAWING ***************** ///
TCanvas * cInputAbsorption = new TCanvas ("cInputAbsorption", "cInputAbsorption", 600, 600);
hInputAbsorption[0]->Draw();
hInputAbsorption[0]->GetXaxis()->SetTitle("Wavelength (nm)");
hInputAbsorption[0]->GetYaxis()->SetTitle("Absorption Length (mm)");
for (int iEn = 1; iEn < NENERGIES; iEn++){
hInputAbsorption[iEn]->Draw("same");
}
gPad->SetGrid();
TCanvas * cDepoEn = new TCanvas ("cDepoEn", "cDepoEn", 600, 600);
hDepoEn[0]->Draw();
hDepoEn[0]->GetXaxis()->SetTitle("Deposited Energy (GeV)");
hDepoEn[0]->GetYaxis()->SetTitle("Counts");
for (int iEn = 1; iEn < NENERGIES; iEn++){
hDepoEn[iEn]->Draw("same");
}
gPad->SetGrid();
int selEn = 0;
TCanvas * cAttEn = new TCanvas ("cAttEn", "cAttEn", 600, 600);
// cAttEn->Divide(2,3);
hAttEn[selEn][0]->Draw();
hAttEn[selEn][0]->GetXaxis()->SetTitle("Measured Energy (GeV)");
hAttEn[selEn][0]->GetYaxis()->SetTitle("Counts");
for (int iAtt = 1; iAtt < nATT; iAtt++){
hAttEn[selEn][iAtt]->Draw("same");
}
gPad->SetGrid();
TCanvas * cResolution = new TCanvas ("cResolution", "cResolution", 600, 600);
grResolution->SetMarkerStyle (21);
grResolution->GetXaxis()->SetTitle("Energy (GeV)");
grResolution->GetYaxis()->SetTitle("#sigma_{E}/E");
grResolution->Draw("ALPE");
gPad->SetGrid();
TCanvas * cLinearity = new TCanvas ("cLinearity", "cLinearity", 600, 600);
grLinearity->SetMarkerStyle (21);
grLinearity->GetXaxis()->SetTitle("Energy (GeV)");
grLinearity->GetYaxis()->SetTitle("Linearity");
grLinearity->GetXaxis()->SetRangeUser(0, 12);
grLinearity->GetYaxis()->SetRangeUser(0, 11);
grLinearity->Draw("ALPE");
gPad->SetGrid();
}
<file_sep>#include "CreateTree.hh"
CreateTree* CreateTree::fInstance = NULL;
CreateTree::CreateTree(TString name, bool energy_fiber, bool init_data, bool pos_fiber, bool opPhotons, bool timing, bool double_ro)
{
if( fInstance )
{
return;
}
this -> ENERGY_FIBER = energy_fiber;
this -> INIT_DATA = init_data;
this -> POS_FIBER = pos_fiber;
this -> OPPHOTONS = opPhotons;
this -> TIMING = timing;
this -> DOUBLE = double_ro;
this -> fInstance = this;
this -> fname = name;
this -> ftree = new TTree(name,name);
this->GetTree()->Branch("Event",&this->Event,"Event/I");
// this->GetTree()->Branch("Tot_phot_cer", &this->Tot_phot_cer, "Tot_phot_cer/F");
// this->GetTree()->Branch("Tot_phot_scint", &this->Tot_phot_scint, "Tot_phot_scint/F");
// this->GetTree()->Branch("Total_delta_world", &this->Total_delta_world, "Total_delta_world/F");
// this->GetTree()->Branch("Total_energy_world", &this->Total_energy_world, "Total_energy_world/F");
// this->GetTree()->Branch("Total_ion_energy_world", &this->Total_ion_energy_world, "Total_ion_energy_world/F");
// this->GetTree()->Branch("Total_nonion_energy_world",&this->Total_nonion_energy_world,"Total_nonion_energy_world/F");
// this->GetTree()->Branch("Total_em_energy",&this->Total_em_energy,"Total_em_energy/F");
if( this -> INIT_DATA )
{
// this->GetTree()->Branch("InitialEnergy",&this->InitialEnergy,"InitialEnergy/F");
this->GetTree()->Branch("InitialPositionX",&this->InitialPositionX,"InitialPositionX/F");
this->GetTree()->Branch("InitialPositionY",&this->InitialPositionY,"InitialPositionY/F");
this->GetTree()->Branch("InitialPositionZ",&this->InitialPositionZ,"InitialPositionZ/F");
// this->GetTree()->Branch("InitalMomentumDirectionX",&this->InitalMomentumDirectionX,"InitalMomentumDirectionX/F");
// this->GetTree()->Branch("InitalMomentumDirectionY",&this->InitalMomentumDirectionY,"InitalMomentumDirectionY/F");
// this->GetTree()->Branch("InitalMomentumDirectionZ",&this->InitalMomentumDirectionZ,"InitalMomentumDirectionZ/F");
}
if( this -> ENERGY_FIBER)
{
this->GetTree()->Branch("Total_energy_dead_material",&this->Total_energy_dead_material,"Total_energy_dead_material/F");
this->GetTree()->Branch("Plastic_tag_ID",&this->Plastic_tag_ID,"Plastic_tag_ID/I");
this->GetTree()->Branch("Total_energy",&this->Total_energy,"Total_energy/F");
this->GetTree()->Branch("Total_ion_energy",&this->Total_ion_energy,"Total_ion_energy/F");
this->GetTree()->Branch("Total_ion_energy_att",&this->Total_ion_energy_att,"Total_ion_energy_att[13]/F");
this->GetTree()->Branch("Total_ion_energy_att_rear",&this->Total_ion_energy_att_rear,"Total_ion_energy_att_rear[13]/F");
this->GetTree()->Branch("Total_ion_energy_att_front",&this->Total_ion_energy_att_front,"Total_ion_energy_att_front[13]/F");
}
if( this -> POS_FIBER)
{
this->GetTree()->Branch("depositionX",&depositionX);
this->GetTree()->Branch("depositionY",&depositionY);
this->GetTree()->Branch("depositionZ",&depositionZ);
this->GetTree()->Branch("Energy_deposited",&Energy_deposited);
this->GetTree()->Branch("Time_deposit",&Time_deposit);
}
if( this -> OPPHOTONS)
{
this->GetTree()->Branch("opPhoton_n",&this->opPhoton_n,"opPhoton_n/I");
// this->GetTree()->Branch("opPhoton_n_type",&this->opPhoton_n_type);
// this->GetTree()->Branch("opPhoton_n_ext",&this->opPhoton_n_ext,"opPhoton_n_ext/I");
// this->GetTree()->Branch("opPhoton_n_ext_type",&this->opPhoton_n_ext_type);
// this->GetTree()->Branch("opPhoton_n_ext_end",&this->opPhoton_n_ext_end);
this->GetTree()->Branch("opPhoton_n_det",&this->opPhoton_n_det,"opPhoton_n_det/I");
// this->GetTree()->Branch("opPhoton_n_det_type",&this->opPhoton_n_det_type);
this->GetTree()->Branch("opPhoton_n_det_end",&this->opPhoton_n_det_end);
// this->GetTree()->Branch("opPhoton_waveLength_det",&opPhoton_waveLength_det);
// this->GetTree()->Branch("opPhoton_time_det",&opPhoton_time_det);
// this->GetTree()->Branch("opPhoton_trackLength_det",&opPhoton_trackLength_det);
}
this->Clear();
}
CreateTree::~CreateTree()
{}
bool CreateTree::Write()
{
TString filename = this->GetName();
filename+=".root";
TFile* file = new TFile(filename,"RECREATE");
this->GetTree()->Write();
file->Write();
file->Close();
return true;
}
void CreateTree::Clear()
{
Event = 0;
/*
Tot_phot_cer = 0;
Tot_phot_scint = 0;*/
/*
Total_delta_world = 0;
Total_energy_world = 0;
Total_ion_energy_world = 0;
Total_nonion_energy_world = 0;*/
if( this->INIT_DATA )
{
InitialEnergy = 0;
InitialPositionX = 0;
InitialPositionY = 0;
InitialPositionZ = 0;
InitalMomentumDirectionX = 0;
InitalMomentumDirectionY = 0;
InitalMomentumDirectionZ = 0;
}
if( this->ENERGY_FIBER )
{
Total_energy_dead_material = 0;
Plastic_tag_ID = -1;
Total_energy = 0;
Total_ion_energy = 0;
for (int j = 0; j< 13; j++)
{
Total_ion_energy_att [j] = 0;
Total_ion_energy_att_rear [j] = 0;
Total_ion_energy_att_front[j] = 0;
}
}
if( this->POS_FIBER )
{
depositionX.clear();
depositionY.clear();
depositionZ.clear();
Energy_deposited.clear();
Time_deposit.clear();
}
if( this->OPPHOTONS )
{
opPhoton_n = 0;
opPhoton_n_type.clear();
opPhoton_n_ext = 0;
opPhoton_n_ext_type.clear();
opPhoton_n_ext_end.clear();
opPhoton_n_det = 0;
opPhoton_n_det_type.clear();
opPhoton_n_det_end.clear();
opPhoton_waveLength_det.clear();
opPhoton_time_det.clear();
opPhoton_trackLength_det.clear();
}
}
<file_sep>ECAL Endcap tapered PbWO4 crystal
==========
Single PbWO4 crystal of CMS EE tapered geometry, read-out using a PMT/VPT like photodetector on the rear face.
Possibility to add a front photodetector to study double-side read-out configuration.
Software allows to study the light collection efficiency inside the crystal as well as the energy resolution and linearity of response to high energy particles by factorizing the processes of:
1. shower development + energy deposition
2. convolution with look-up table of light collection efficiency curves for different levels of radiation damage
Effects of containment and beam spot size can also be investigated.
| 259ef536f373ed71757c3abe77f87b685dffff5d | [
"Markdown",
"C",
"C++"
] | 3 | C | marco-toli/ECAL_Endcap_tapered | 62879ee2c9705d48389d787983cb82c7012d2543 | 0d406fd3bd335c710b4a690ba9660f14c72ca6b2 | |
refs/heads/master | <repo_name>EpitechAntoineMasson/Global-game-Jam<file_sep>/GGJ/Assets/Script/GameController.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
public class GameController : MonoBehaviour
{
public TextMesh restartText;
public TextMesh gameOverText;
private bool gameOver;
private bool restart;
void Start()
{
gameOver = false;
restart = false;
restartText.text = "";
gameOverText.text = "";
}
void Update()
{
if (restart)
{
if (Input.GetKeyDown(KeyCode.R))
{
SceneManager.LoadScene ("GGJ game");
}
}
}
void FixedUpdate()
{
if (gameOver)
{
restartText.text = "Press 'R' for Restart";
restart = true;
}
}
public void GameOver()
{
gameOverText.text = "Game Over!";
gameOver = true;
}
}
<file_sep>/GGJ/Assets/Script/ChangeColor.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ChangeColor : MonoBehaviour {
public Material matofobject;
public KeyCode colo1;
public KeyCode colo2;
public KeyCode colo3;
public KeyCode colo4;
void Update () {
if (Input.GetKeyDown (colo3)) {
matofobject.color = Color.blue;
} else if (Input.GetKeyDown (colo1)) {
matofobject.color = Color.green;
} else if (Input.GetKeyDown (colo4)) {
matofobject.color = Color.yellow;
} else if (Input.GetKeyDown (colo2)) {
matofobject.color = Color.red;
}
}
}
<file_sep>/GGJ/Assets/Script/MenuPause.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class MenuPause : MonoBehaviour {
public KeyCode input;
private bool isPaused = false;
public GameObject canvas;
public GameObject scoreCanvas;
// Update is called once per frame
void Update () {
if (Input.GetKeyDown(input))
isPaused = !isPaused;
if (isPaused)
{
Time.timeScale = 0f;
canvas.SetActive(true);
scoreCanvas.SetActive(false);
}
else
{
Time.timeScale = 1.0f;
canvas.SetActive(false);
scoreCanvas.SetActive(true);
}
}
}
<file_sep>/GGJ/Assets/Script/RandSpawn.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
public class RandSpawn : MonoBehaviour {
public Vector3[] positions;
public GameObject canvas;
public GameObject scoreCanvas;
public AudioSource m_MyAudioSource;
void Start()
{
int randomNumber = Random.Range(0, positions.Length);
transform.position = positions[randomNumber];
}
void Update()
{
if (Input.GetKey("escape"))
Application.Quit();
}
void OnTriggerEnter(Collider other)
{
if (other.tag == "Player")
{
//canvas.SetActive(true);
//Destroy(gameObject); Debug.log
//scoreCanvas.SetActive(false);
//Destroy(other.gameObject);
//Time.timeScale = 0f;
//Application.Quit();
SceneManager.LoadScene(SceneManager.GetActiveScene().buildIndex + 1);
}
if (other.tag == "Zic")
{
m_MyAudioSource.Play();
}
if (other.tag == "Wall")
{
int randomNumber = Random.Range(0, positions.Length);
transform.position = positions[randomNumber];
}
}
} | 9a7a087c06b66a491c569f8dbbd6b473ab0097f5 | [
"C#"
] | 4 | C# | EpitechAntoineMasson/Global-game-Jam | 0011b1f0f88009e603baeb042993e5881f4290f9 | 1b96ab1be49b7a777a93396ded019b27aaf877cc | |
refs/heads/main | <repo_name>ako2cjairo1/expense-tracker<file_sep>/src/components/Main/List/List.jsx
import React, { useContext } from 'react'
import { List as MUIList, ListItem, ListItemAvatar, ListItemText, Avatar, ListItemSecondaryAction, IconButton, Slide, Typography } from "@material-ui/core"
import { Delete, MoneyOff } from "@material-ui/icons"
import { ExpenseTrackerContext } from "../../../context/context"
import useStyles from "./styles"
import formatAmount from "../../../utils/formatAmount"
export default function List() {
const classes = useStyles()
const { transactions, deleteTransaction } = useContext(ExpenseTrackerContext)
const itemDetails = (type, amount, date) => {
const formattedAmount = formatAmount(type === "Income" ? amount : amount * -1)
return (
<div style={{ display: "flex", justifyContent: "space-between" }}>
<div>P{formattedAmount}</div>
<div>{date}</div>
</div>
)
}
if(transactions && transactions.length <= 0) {
return (
<Typography variant="subtitle1" align="center" style={{ lineHeight: "1.5em", marginTop: "20px" }}>
- No Transactions -
</Typography>
)
}
return (
<MUIList dense={false} className={classes.list}>
{transactions.map(({ id, type, category, amount, date }) => (
<Slide direction="down" in mountOnEnter unmountOnExit key={id}>
<ListItem>
<ListItemAvatar>
<Avatar className={type === "Income" ? classes.avatarIncome : classes.avatarExpense }>
<MoneyOff />
</Avatar>
</ListItemAvatar>
<ListItemText primary={category} secondary={itemDetails(type, amount, date)} />
<ListItemSecondaryAction>
<IconButton edge="end" aria-label="delete" onClick={() => deleteTransaction(id)}>
<Delete />
</IconButton>
</ListItemSecondaryAction>
</ListItem>
</Slide>
))}
</MUIList>
)
}
<file_sep>/src/utils/formatDate.js
export default function formatDate(date) {
const newDate = new Date(date)
let month = `${newDate.getMonth() + 1}`
let day = `${newDate.getDate()}`
const year = newDate.getFullYear()
if(month.length < 2) month = `0${month}`
if(day.length < 2) day = `0${day}`
return [year, month, day].join("-")
} | 43c546f0646bbce1a505a8d2a936ec8e10c8f7c0 | [
"JavaScript"
] | 2 | JavaScript | ako2cjairo1/expense-tracker | 0e947570ec6dc5d727371993f3117e023e255029 | e23cdecd7cc106fdc0816c7f6c2afdb259bb7c31 | |
refs/heads/master | <file_sep>#include "Date.h"
#include<iostream>
using namespace std;
void Date::set_day(int day) {
if (day < 1 || day>31)
day = 1;
this->day = day;
}
void Date::set_month(int month) {
if (month < 1 || month>12)
month = 1;
this->month = month;
}
void Date::set_year(int year) {
if (year < 2020||year>=2022)
year = 2020;
this->year = year;
}
Date::Date() {
day = 4;
month = 1;
year = 2020;
}
Date::Date(int day, int month, int year) {
set_day(day);
set_month(month);
set_year(year);
}
bool Date::operator==(const Date& other) const {
return ((this->day == day) && (this->month == month) && (this->year == year));
}
void Date::deserialize(ifstream& ifs) {
if (!ifs.is_open()) {
cout << "Can not open this file!\n";
return;
}
ifs.read((char*)&day, sizeof(day));
ifs.read((char*)&month, sizeof(month));
ifs.read((char*)&year, sizeof(year));
if (ifs.good()) {
cout << "Successfully deserialized!\n";
return;
}
else
cout << "Failed\n";
}
void Date::serialize(ofstream& ofs) const {
ofs.write((const char*)&day, sizeof(day));
ofs.write((const char*)&month, sizeof(month));
ofs.write((const char*)&year, sizeof(year));
}
ostream& operator<<(ostream& os, const Date& obj) {
obj.print(os);
return os << endl;
}
void Date::print(ostream& os) const {
os << year;
os << "/";
os << setw(2);
os << setfill('0');
os << month;
os << "/";
os << day;
}
int Date::compare_to(const Date& other) const
{
if (get_day() > other.get_day())
return -1;
else if (get_day() < other.get_day())
{
return 1;
}
else
{
if (get_month() > other.get_month())
return -1;
else if (get_month() < other.get_month())
return 1;
else
{
if (get_year() > other.get_year())
return -1;
else if (get_year() < other.get_year())
return 1;
else
return 0;
}
}
}
/*bool Date::is_leap_year(int year) {
return((year % 4 == 0 && year % 100 != 0) || (year % 400 == 0));
}
void Date::optional(int n) {
this->day += n;
int* this_year = nullptr;
if (is_leap_year(year)) {
this_year = leap;
}
else
this_year = not_leap;
if (day > this_year[month]) {
day -= this_year[month];
++month;
}
if (month > 12) {
month = 1;
++year;
}
}*/
<file_sep>#include"RoomsList.h"
void RoomsList::Resize() {
Room* temp = new Room[capacity*2];
for (int i = 0; i < size; i++)
temp[i] = rooms[i];
delete[] rooms;
rooms = temp;
}
void RoomsList::Copy_From(const RoomsList& other) {
rooms = new Room[other.capacity];
for (int i = 0; i < other.size; i++)
rooms[i] = other.rooms[i];
capacity = other.capacity;
size = other.size;
}
void RoomsList::Free() {
delete[] rooms;
}
RoomsList::RoomsList() {
capacity = 4;
rooms = new Room[capacity];
size = 0;
}
RoomsList::RoomsList(int new_capacity) {
if (capacity == 0) {
capacity = 1;
}
rooms = new Room[new_capacity];
size = 0;
capacity = new_capacity;
}
RoomsList::RoomsList(const RoomsList& other) {
Copy_From(other);
}
RoomsList& RoomsList::operator=(const RoomsList& other) {
if (this != &other) {
Free();
Copy_From(other);
}
return *this;
}
RoomsList::~RoomsList() {
Free();
}
void RoomsList::push_back(const Room& new_room) {
if (!is_valid_room_number(new_room.get_room_num()))
return;
if (is_full())
Resize();
rooms[size++] = new_room;
}
void RoomsList::shift_left(int index)
{
for (int i = index; i < size - 1; i++)
{
rooms[i] = rooms[i + 1];
}
}
int RoomsList::remove_at(int index)
{
if (size == 0 || index > size)
{
return 0;
}
shift_left(index);
size--;
return 1;
size--;
}
Room& RoomsList::operator[](int index) const {
return rooms[index];
}
ostream& operator<<(ostream& os, RoomsList& obj) {
obj.print(os);
return os << endl;
}
void RoomsList::print(ostream& os) const {
for (int i = 0; i < size; i++) {
rooms[i].print();
}
}
void RoomsList::serialize(ofstream& ofs) const {
if (!ofs.is_open()) { return;}
ofs.write((const char*)&size, sizeof(size));
ofs.write((const char*)&capacity, sizeof(capacity));
for (int i = 0; i < size; i++) {
rooms[i].serialize(ofs);
}
}
void RoomsList::deserialize(ifstream& ifs) {
if (!ifs.is_open()) { return; }
ifs.read((char*)&size, sizeof(size));
ifs.read((char*)&capacity, sizeof(capacity));
int len;
ifs.read((char*)&len, sizeof(int));
for (int i = 0; i < len; i++) {
rooms[i].deserialize(ifs);
push_back(rooms[i]);
}
}
<file_sep>#include"Hotel.h"
int main() {
Hotel::i().run();
return 0;
}<file_sep>#ifndef HOTEL_H
#define HOTEL_H
#include"Date.h"
#include"RoomsList.h"
#include"Room.h"
#include"Reservation.h"
using namespace std;
class Hotel {
private:
RoomsList rooms;
Hotel();
Room* get_room(int room_num) const;
Room* get_unavailable_rooms(int from_day, int from_month, int from_year,int to_day, int to_month, int to_year);
void display();
public:
static Hotel& i();
int first_free_index();
bool contains_room(unsigned room_num) const { return get_room(room_num); };
bool create_reservation(const Reservation& res);
void run();
bool serialize() const;
bool deserialize();
};
#endif // !HOTEL_H<file_sep>#include"Reservation.h"
bool has_digits(const char* str, int len)
{
for (int i = 0; i < len; i++)
{
if (str[i] >= '0' && str[i] <= '9')
return true;
}
return false;
}
void Reservation::Copy_From(const Reservation& other) {
note = new char[strlen(other.note) + 1];
strcpy(note, other.note);
guests = other.guests;
breakfast = other.breakfast;
}
void Reservation::Free() {
delete[] note;
note = nullptr;
}
Reservation::Reservation(int room_num, int from_day, int from_month, int from_year, int to_day, int to_month, int to_year,
const char* new_note, unsigned beds, int new_guests, bool new_breakfast)
: Room(room_num,from_day,from_month,from_year,to_day,to_month,to_year,beds),
note(new char[strlen(new_note) + 1]), guests(new_guests), breakfast(new_breakfast) {}
Reservation::Reservation(const Reservation& other) {
Copy_From(other);
}
Reservation& Reservation::operator=(const Reservation& other) {
if (this != &other) {
Free();
Copy_From(other);
}
return *this;
}
Reservation::~Reservation() {
Free();
}
void Reservation::set_note(const char* note)
{
//delete[] note;
int len = strlen(note);
if (has_digits(note, len))
{
this->note[0] = '\0';
return;
}
this->note = new char[len + 1];
strcpy(this->note, note);
}
void Reservation::deserialize(ifstream& ifs) {
if (!ifs.is_open()) {
cout << "Can not open this file!\n";
return;
}
size_t len;
ifs.read((char*)&len, sizeof(len));
note = new char[len+1];
if (note) {
ifs.read(note, len);
note[len] = '\0';
}
ifs.read((char*)&guests, sizeof(guests));
ifs.read((char*)&breakfast, sizeof(breakfast));
if (ifs.good()) {
cout << "Successfully deserialized!\n";
}
else
cout << "Failed\n";
}
void Reservation::serialize(ofstream& out) const {
size_t len = strlen(note) + 1;
out.write((const char*)&len, sizeof(len));
out.write((const char*)note, len);
out.write((const char*)&guests, sizeof(guests));
out.write((const char*)&breakfast, sizeof(breakfast));
}
void Reservation::print(ostream& os) const {
Room::print(os);
os << "The room has reserved by: " << note << " with "
<< guests << " guest(s)." << endl;
if (breakfast) cout << get_price() << " EUR for breakfast.\n";
}
ostream& operator<<(ostream& os, const Reservation& r) {
r.print(os);
return os << endl;
}
<file_sep># Hotel
Project in Sofia University, Object-Oriented-Programming Course.
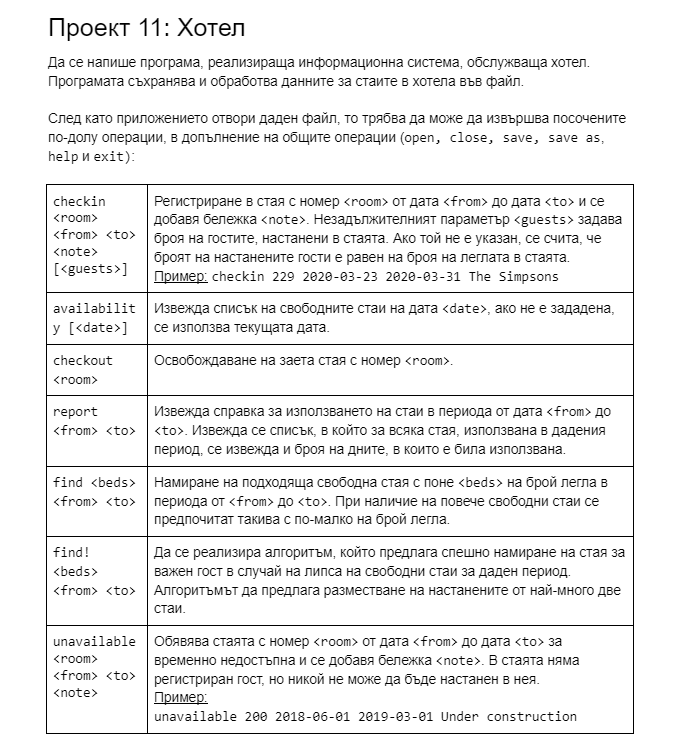
<file_sep>#ifndef ROOMSLIST_H
#define ROOMSLIST_H
#define _CRT_SECURE_NO_WARNINGS
#include"Room.h"
using namespace std;
class RoomsList {
private:
Room* rooms;
int size;
int capacity;
void Resize();
void Copy_From(const RoomsList& other);
void Free();
public:
RoomsList();
RoomsList(int new_capacity);
RoomsList(const RoomsList& other);
RoomsList& operator=(const RoomsList& other);
~RoomsList();
void push_back(const Room& room);
void shift_left(int index);
int remove_at(int index);
int get_size() const { return size; };
int get_capacity() const { return capacity; };
bool is_full() { return size == capacity; };
bool is_valid_room_number(int room_number) { return (room_number >= 1 && room_number <= 50); };
Room& operator[](int index) const;
void serialize(ofstream& ofs) const;
void deserialize(ifstream& ifs);
friend ostream& operator<<(ostream& os, const RoomsList& obj);
void print(ostream& os=cout) const;
};
#endif // !ROOMSLIST_H<file_sep>#include"Room.h"
Room::Room() : from_date(4,1,2020), to_date(4,1,2021), is_init(false) , beds(0),room_num(0) {}
Room::Room(int new_room_num, int from_day, int from_month, int from_year, int to_day, int to_month, int to_year, unsigned new_beds)
:from_date(from_day,from_month,from_year),to_date(to_day,to_month,to_year) , room_num(new_room_num),beds(new_beds)
{
if (!is_valid_date())
to_date = from_date;
}
void Room::deserialize(ifstream& ifs) {
if (!ifs.is_open()) {
cout << "Can not open this file!\n";
return;
}
ifs.read((char*)&from_date, sizeof(from_date));
ifs.read((char*)&to_date, sizeof(to_date));
ifs.read((char*)&room_num, sizeof(room_num));
ifs.read((char*)&beds, sizeof(beds));
if (ifs.good()) {
cout << "Successfully deserialized!\n";
}
else
cout << "Failed\n";
}
void Room::serialize(ofstream& out) const
{
out.write((const char*)&from_date, sizeof(from_date));
out.write((const char*)&to_date, sizeof(to_date));
out.write((const char*)&room_num, sizeof(room_num));
out.write((const char*)&beds, sizeof(beds));
/*const char* n_date = from_date.get_date();
int len = strlen(n_date)+1;
out.write((const char*)&len, sizeof(len));
out.write(n_date, len);
n_date = to_date.get_date();
len = strlen(n_date) + 1;
out.write((const char*)&len, sizeof(len));
out.write(n_date, len);
*/
}
void Room::print(ostream& os) const {
os << "Room number: " << room_num << endl;
os << "From date: "; from_date.print();
os <<' '<<"To date: "; to_date.print();
}
ostream& operator<<(ostream& os, const Room& obj) {
obj.print(os);
return os << endl;
}<file_sep>#ifndef RESERVATION_H
#define RESERVATION_H
#include"Room.h"
#include<fstream>
#include<iostream>
using namespace std;
class Reservation : public Room {
private:
char* note;
int guests;
bool breakfast;
void Copy_From(const Reservation& other);
void Free();
public:
Reservation(int room_num=0, int from_day=4, int from_month=1, int from_year=2020, int to_day=4, int to_month=1, int to_year=2021,
const char* new_note="<Unnamed>", unsigned beds=0, int new_guests=0,bool breakfast=false);
Reservation(const Reservation& other);
Reservation& operator=(const Reservation& other);
~Reservation();
const char* get_note() const { return note; };
void set_note(const char* note);
int get_guests() const { return guests; };
double get_price() const { return 9.9; };
void serialize(ofstream& ofs) const;
void deserialize(ifstream& ifs);
void print(ostream& os=cout) const;
friend ostream& operator<<(ostream& os, const Reservation& obj);
};
#endif // !RESERVATION_H
<file_sep>#ifndef ROOM_H
#define ROOM_H
#define _CRT_SECURE_NO_WARNINGS
#include"Date.h"
#include<iostream>
#include <fstream>
using namespace std;
class Room {
private:
Date from_date;
Date to_date;
bool is_init;
int room_num;
unsigned beds;
public:
Room();
Room(int new_room_num,int from_day,int from_month,int from_year,int to_day,int to_month,int to_year, unsigned new_beds);
const Date& get_from_date() const { return from_date; };
const Date& get_to_date() const { return to_date; };
int get_room_num() const { return room_num; };
unsigned get_beds() const { return beds; };
bool get_is_init() const { return is_init; };
bool is_valid_date() { return from_date.compare_to(to_date) >= 0; };
void serialize(ofstream& out) const;
void deserialize(ifstream& ifs);
void print(ostream& os=cout) const;
friend ostream& operator<<(ostream& os, const Room& obj);
/* void set_total_beds(int new_beds);
int get_free_beds() const { return total_beds - reserved_beds; };
int get_total_beds() const { return total_beds; }
int get_reserved_beds() const { return reserved_beds; }*/
};
#endif // !ROOM_H
<file_sep>#ifndef DATE_H
#define DATE_H
#include<iomanip>
#include<iostream>
#include <fstream>
using namespace std;
class Date {
private:
int day;
int month;
int year;
public:
void set_day(int);
void set_month(int);
void set_year(int);
int get_day() const { return day; };
int get_month() const { return month; };
int get_year() const { return year; };
Date();
Date(int day, int month, int year);
int compare_to(const Date& other) const;
bool operator==(const Date& other) const;
//bool is_leap_year(int year);
//void optional(int n);
friend ostream& operator<<(ostream& os, const Date& obj);
virtual void print(ostream& os=cout) const;
void serialize(ofstream& ofs) const;
void deserialize(ifstream& ifs);
};
#endif<file_sep>#include"Hotel.h"
#include<fstream>
#include<cstring>
using namespace std;
const char* SAVE_FILE_NAME = "hotel_system.bin";
const size_t MAX_INPUT_LEN = 128;
Hotel::Hotel(){}
int Hotel::first_free_index() {
for (int i = 0; i < rooms.get_size(); i++) {
if (&rooms[i] == nullptr)
return i;
}
return -1;
}
Room* Hotel::get_room(int room_num) const
{
for (int i = 0; i < rooms.get_size(); i++) {
if (rooms[i].get_room_num() == room_num)
return &rooms[i];
}
return nullptr;
}
bool Hotel::create_reservation(const Reservation& res) {
if (get_room(res.get_room_num()) == nullptr) {
rooms.push_back(res);
return true;
}
return false;
}
Room* Hotel::get_unavailable_rooms(int from_day,int from_month,int from_year,
int to_day,int to_month,int to_year) {
Date from_date(from_day, from_month, from_year);
Date to_date(to_day, to_month, to_year);
for (int i = 0; i < rooms.get_size(); i++) {
if (from_date == rooms[i].get_from_date() && to_date == rooms[i].get_to_date())
return &rooms[i];
}
return nullptr;
/*for (int i = 0; i < rooms.get_size(); i++) {
if((rooms[i].get_from_date().compare_to(from_date)) == 0 && (rooms[i].get_to_date().compare_to(to_date))== 0)
return &rooms[i];
}*/
}
Hotel& Hotel::i()
{
static Hotel h;
return h;
}
bool Hotel::serialize() const {
ofstream ofs;
ofs.open(SAVE_FILE_NAME, ios::binary);
if (!ofs) {
cout << "Can not open " << SAVE_FILE_NAME << "file for writing!\n";
return false;
}
int len = rooms.get_size();
ofs.write((const char*)&len, sizeof(len));
for (int i = 0; i < len; i++)
rooms[i].serialize(ofs);
ofs.close();
return true;
}
bool Hotel::deserialize() {
ifstream ifs;
ifs.open(SAVE_FILE_NAME, ios::binary);
if (!ifs.is_open()) return false;
int len=rooms.get_size();
ifs.read((char*)&len, sizeof(len));
for (int i = 0; i < len; i++)
rooms.deserialize(ifs);
ifs.close();
return true;
}
void Hotel::run() {
if (deserialize()) cout << "Successfully loaded " << rooms.get_size() << " room(s)" << endl;
else
cout << "No previous hotel system information.\n";
cout << endl;
display();
cout << endl;
char command[MAX_INPUT_LEN];
char note[MAX_INPUT_LEN];
int number;
int from_day, from_month, from_year;
int to_day, to_month, to_year;
do {
cout << "ENTER AN OPTION: "; cin >> command;
if (strcmp(command, "help") == 0) {
display();
}
else if (strcmp(command, "check_in") == 0) {
cout << "****CHECK-IN****\n";
cout << "Room number: "; cin >> number;
cout << "From date: "; cin >> from_day>> from_month>> from_year;
cout << "To Date: "; cin >> to_day >> to_month>> to_year;
cout << "Note: "; cin >> note;
if (create_reservation({number,from_day,from_month,from_year,to_day, to_month, to_year,note})) {
cout << "Room added successfully!\n";
}
else {
cout << "Room " << number << " already exists!\n";
}
cout << endl;
}
else if (strcmp(command, "extras") == 0) {
cout << "****ADDITIONAL EXTRAS FOR YOUR ROOM****\n";
cout << "Enter your room number: ";
cin >> number;
bool breakfast;
if (contains_room(number)) {
cout << "Do you want breakfast? Enter: 1 for YES or 0 for NO: "; cin >> breakfast;
if (breakfast) {
create_reservation({ number, breakfast });
cout << "Successfully added breakfast to your reservation!\n";
}
}
else
cout << "Sorry, you have to check-in first!\n";
cout << endl;
}
else if (strcmp(command, "available_rooms") == 0) {
cout << "****LISTS OF AVAILABLE ROOMS****\n";
cout << "From date: "; cin >> from_day >> from_month >> from_year;
cout << "To date: "; cin >> to_day >> to_month >> to_year;
Room* res = get_unavailable_rooms(from_day, from_month, from_year, to_day, to_month, to_year);
if (res) { cout << *res << endl;
return;
}
else
cout << "There are not available rooms on this dates!\n";
cout << endl;
}
else if (strcmp(command, "check_out") == 0) {
cout << "Enter your room number: ";
cin >> number;
if(contains_room(number)){
for (int i = 0; i < rooms.get_size(); i++)
rooms.remove_at(number);
cout << "Room with number: " << number << " checked-out successfully!\n";
}
else {
cout << "Room " << number << " not found! \n";
}
cout << endl;
}
else if (strcmp(command, "report_rooms") == 0) {
cout << "****LISTS OF RESERVED ROOMS****\n";
cout << "From date: "; cin >> from_day >> from_month >> from_year;
cout << "To date: "; cin >> to_day >> to_month >> to_year;
Room* res = get_unavailable_rooms(from_day, from_month, from_year, to_day, to_month, to_year);
for (int i = 0; i < rooms.get_size(); i++) {
if(res) {
rooms[i].print();
cout << endl;
}
else
cout << "Room from the period: " << rooms[i].get_from_date() << " - " << rooms[i].get_to_date() << " not found!\n";
}
cout << endl;
}
else if (strcmp(command, "find_room") == 0) {
}
else if (strcmp(command, "important_guest") == 0) {
}
else if (strcmp(command, "unavailable_room") == 0) {
}
else if (strcmp(command, "exit") == 0) {
if (serialize())
cout << "Successfully saved the system information!\n";
else {
cout << "Couldn't saved the system information. Exit anyway: [y/n]? : ";
char option;
cin.ignore(MAX_INPUT_LEN, '\n');
cin.get(option);
if (option == 'y' || option == 'n')
command[0] = '\n';
}
}
else {
cout << "Unknown command! Type 'help' for more information: " << endl;
}
} while (strcmp(command, "exit") != 0);
}
void Hotel::display() {
cout << "*********** HOTEL JOANNNA MANAGEMENT SYSTEM ***********" << endl;
cout << "help - Prints this information." << endl;
cout << "check_in - Check-In Room" << endl;
cout << "extras - Additional EXTRAS for the room" << endl;
cout << "available_rooms - Available rooms" << endl;
cout << "check_out - Chechk-Out Room" << endl;
cout << "report_rooms - Show report of all used rooms" << endl;
cout << "find_room - Find suitable room with a certain number of beds" << endl;
cout << "important_guest - Find room for important guest" << endl;
cout << "unavailable_room - Show rooms which are unavailables to use" << endl;
cout << "exit - saves the system and exit the program" << endl;
}
| d439c1139bf70bc672b069adc33691b4def8aca4 | [
"Markdown",
"C++"
] | 12 | C++ | joannatufkova/Hotel | ae123df0be900053d66a8bcf705a34f573b80395 | 439ddcc4336b1230300f4eaf1e212beb4387a53b | |
refs/heads/master | <repo_name>kayacanv/twitter-auto-rt-bot<file_sep>/app.js
const express = require('express');
const app = express();
app.get("/", (req,res)=>{
var Twit = require('twit');
var key = require('./key.json');
let T = new Twit(key)
let our_id=""
let our_username="nocontextnocon2"
function request(url){
return T.get(url).then( data => {
return data;
});
}
async function getMax(id){
let mxFav = -1;
let tweetId = "";
let url = "https://api.twitter.com/1.1/statuses/user_timeline.json?user_id=" + id + "&count=200"+"&stringify_ids=true";
let tweets = await request(url);
tweets = tweets.data;
tweets.forEach(async tweet => {
let str = tweet.created_at;
let created_date = new Date(
str.replace(/^\w+ (\w+) (\d+) ([\d:]+) \+0000 (\d+)$/,
"$1 $2 $4 $3 UTC"));
created_date = created_date.getTime();
let now = new Date();
now = now.getTime();
let hours = 3;
if(now - created_date > hours*60*60*1000) // in the last x hours
return ;
let fav = 0;
if(tweet.retweeted_status == undefined)
fav = tweet.favorite_count;
else
fav = tweet.retweeted_status.favorite_count; //rts included
if(fav > mxFav){
mxFav = fav;
tweetId = tweet.id_str;
}
});
return [mxFav,tweetId];
}
async function asyncForEach(array, callback) {
for (let index = 0; index < array.length; index++) {
await callback(array[index], index, array);
}
}
async function autoRT(id , count=200)
{
let url="";
if(id => /\D/.test(id))
url = "https://api.twitter.com/1.1/friends/ids.json?cursor=-1&screen_name="+id+"&count="+count+"&stringify_ids=true";
else
url = "https://api.twitter.com/1.1/friends/ids.json?cursor=-1&user_id="+id+"&count="+count+"&stringify_ids=true";
let users = await request(url);
users = users.data.ids;
let globalMaxFav = -1;
let tweetId = "";
await asyncForEach(users, async id=>{
let data = await getMax(id);
if(data[0] > globalMaxFav){
globalMaxFav = data[0];
tweetId = data[1];
}
console.log(data[0]);
});
console.log(globalMaxFav,tweetId);
T.post('statuses/retweet/:id', { id: tweetId }, (err, data, response)=>{
console.log(data)
});
}
autoRT(our_username);
setInterval( ()=>autoRT(our_username) , 180 * 60 * 1000) // each 180 minutes
res.send("<h1>hi bro</h1>");
});
app.listen(process.env.PORT || 3000, "0.0.0.0");
<file_sep>/README.md
# twitter-auto-rt-bot
twitter rt bot that automatically retweets in every 3 hours by the highest like number
| e61fd145918eae9a03dc1d0fbb3f8edfb14771db | [
"JavaScript",
"Markdown"
] | 2 | JavaScript | kayacanv/twitter-auto-rt-bot | f970f97b7dba9d3efa37c0c97e6436d7e07a4213 | 2802d07624c1e1785c60b68ac86fa0430f7e64bc |
Subsets and Splits
No saved queries yet
Save your SQL queries to embed, download, and access them later. Queries will appear here once saved.