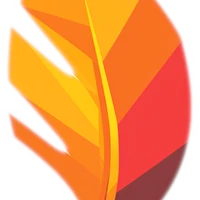
forge1825/FStudent
Updated
•
1
code
stringlengths 26
870k
| docstring
stringlengths 1
65.6k
| func_name
stringlengths 1
194
| language
stringclasses 1
value | repo
stringlengths 8
68
| path
stringlengths 5
194
| url
stringlengths 46
254
| license
stringclasses 4
values |
---|---|---|---|---|---|---|---|
def attr_chain(obj, attr):
"""Follow an attribute chain.
If you have a chain of objects where a.foo -> b, b.foo-> c, etc,
use this to iterate over all objects in the chain. Iteration is
terminated by getattr(x, attr) is None.
Args:
obj: the starting object
attr: the name of the chaining attribute
Yields:
Each successive object in the chain.
"""
next = getattr(obj, attr)
while next:
yield next
next = getattr(next, attr) | Follow an attribute chain.
If you have a chain of objects where a.foo -> b, b.foo-> c, etc,
use this to iterate over all objects in the chain. Iteration is
terminated by getattr(x, attr) is None.
Args:
obj: the starting object
attr: the name of the chaining attribute
Yields:
Each successive object in the chain. | attr_chain | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_util.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_util.py | MIT |
def in_special_context(node):
""" Returns true if node is in an environment where all that is required
of it is being iterable (ie, it doesn't matter if it returns a list
or an iterator).
See test_map_nochange in test_fixers.py for some examples and tests.
"""
global p0, p1, p2, pats_built
if not pats_built:
p0 = patcomp.compile_pattern(p0)
p1 = patcomp.compile_pattern(p1)
p2 = patcomp.compile_pattern(p2)
pats_built = True
patterns = [p0, p1, p2]
for pattern, parent in zip(patterns, attr_chain(node, "parent")):
results = {}
if pattern.match(parent, results) and results["node"] is node:
return True
return False | Returns true if node is in an environment where all that is required
of it is being iterable (ie, it doesn't matter if it returns a list
or an iterator).
See test_map_nochange in test_fixers.py for some examples and tests. | in_special_context | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_util.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_util.py | MIT |
def is_probably_builtin(node):
"""
Check that something isn't an attribute or function name etc.
"""
prev = node.prev_sibling
if prev is not None and prev.type == token.DOT:
# Attribute lookup.
return False
parent = node.parent
if parent.type in (syms.funcdef, syms.classdef):
return False
if parent.type == syms.expr_stmt and parent.children[0] is node:
# Assignment.
return False
if parent.type == syms.parameters or \
(parent.type == syms.typedargslist and (
(prev is not None and prev.type == token.COMMA) or
parent.children[0] is node
)):
# The name of an argument.
return False
return True | Check that something isn't an attribute or function name etc. | is_probably_builtin | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_util.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_util.py | MIT |
def find_indentation(node):
"""Find the indentation of *node*."""
while node is not None:
if node.type == syms.suite and len(node.children) > 2:
indent = node.children[1]
if indent.type == token.INDENT:
return indent.value
node = node.parent
return "" | Find the indentation of *node*. | find_indentation | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_util.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_util.py | MIT |
def find_root(node):
"""Find the top level namespace."""
# Scamper up to the top level namespace
while node.type != syms.file_input:
node = node.parent
if not node:
raise ValueError("root found before file_input node was found.")
return node | Find the top level namespace. | find_root | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_util.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_util.py | MIT |
def does_tree_import(package, name, node):
""" Returns true if name is imported from package at the
top level of the tree which node belongs to.
To cover the case of an import like 'import foo', use
None for the package and 'foo' for the name. """
binding = find_binding(name, find_root(node), package)
return bool(binding) | Returns true if name is imported from package at the
top level of the tree which node belongs to.
To cover the case of an import like 'import foo', use
None for the package and 'foo' for the name. | does_tree_import | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_util.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_util.py | MIT |
def is_import(node):
"""Returns true if the node is an import statement."""
return node.type in (syms.import_name, syms.import_from) | Returns true if the node is an import statement. | is_import | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_util.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_util.py | MIT |
def touch_import(package, name, node):
""" Works like `does_tree_import` but adds an import statement
if it was not imported. """
def is_import_stmt(node):
return (node.type == syms.simple_stmt and node.children and
is_import(node.children[0]))
root = find_root(node)
if does_tree_import(package, name, root):
return
# figure out where to insert the new import. First try to find
# the first import and then skip to the last one.
insert_pos = offset = 0
for idx, node in enumerate(root.children):
if not is_import_stmt(node):
continue
for offset, node2 in enumerate(root.children[idx:]):
if not is_import_stmt(node2):
break
insert_pos = idx + offset
break
# if there are no imports where we can insert, find the docstring.
# if that also fails, we stick to the beginning of the file
if insert_pos == 0:
for idx, node in enumerate(root.children):
if (node.type == syms.simple_stmt and node.children and
node.children[0].type == token.STRING):
insert_pos = idx + 1
break
if package is None:
import_ = Node(syms.import_name, [
Leaf(token.NAME, "import"),
Leaf(token.NAME, name, prefix=" ")
])
else:
import_ = FromImport(package, [Leaf(token.NAME, name, prefix=" ")])
children = [import_, Newline()]
root.insert_child(insert_pos, Node(syms.simple_stmt, children)) | Works like `does_tree_import` but adds an import statement
if it was not imported. | touch_import | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_util.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_util.py | MIT |
def find_binding(name, node, package=None):
""" Returns the node which binds variable name, otherwise None.
If optional argument package is supplied, only imports will
be returned.
See test cases for examples."""
for child in node.children:
ret = None
if child.type == syms.for_stmt:
if _find(name, child.children[1]):
return child
n = find_binding(name, make_suite(child.children[-1]), package)
if n: ret = n
elif child.type in (syms.if_stmt, syms.while_stmt):
n = find_binding(name, make_suite(child.children[-1]), package)
if n: ret = n
elif child.type == syms.try_stmt:
n = find_binding(name, make_suite(child.children[2]), package)
if n:
ret = n
else:
for i, kid in enumerate(child.children[3:]):
if kid.type == token.COLON and kid.value == ":":
# i+3 is the colon, i+4 is the suite
n = find_binding(name, make_suite(child.children[i+4]), package)
if n: ret = n
elif child.type in _def_syms and child.children[1].value == name:
ret = child
elif _is_import_binding(child, name, package):
ret = child
elif child.type == syms.simple_stmt:
ret = find_binding(name, child, package)
elif child.type == syms.expr_stmt:
if _find(name, child.children[0]):
ret = child
if ret:
if not package:
return ret
if is_import(ret):
return ret
return None | Returns the node which binds variable name, otherwise None.
If optional argument package is supplied, only imports will
be returned.
See test cases for examples. | find_binding | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_util.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_util.py | MIT |
def _is_import_binding(node, name, package=None):
""" Will reuturn node if node will import name, or node
will import * from package. None is returned otherwise.
See test cases for examples. """
if node.type == syms.import_name and not package:
imp = node.children[1]
if imp.type == syms.dotted_as_names:
for child in imp.children:
if child.type == syms.dotted_as_name:
if child.children[2].value == name:
return node
elif child.type == token.NAME and child.value == name:
return node
elif imp.type == syms.dotted_as_name:
last = imp.children[-1]
if last.type == token.NAME and last.value == name:
return node
elif imp.type == token.NAME and imp.value == name:
return node
elif node.type == syms.import_from:
# str(...) is used to make life easier here, because
# from a.b import parses to ['import', ['a', '.', 'b'], ...]
if package and str(node.children[1]).strip() != package:
return None
n = node.children[3]
if package and _find("as", n):
# See test_from_import_as for explanation
return None
elif n.type == syms.import_as_names and _find(name, n):
return node
elif n.type == syms.import_as_name:
child = n.children[2]
if child.type == token.NAME and child.value == name:
return node
elif n.type == token.NAME and n.value == name:
return node
elif package and n.type == token.STAR:
return node
return None | Will reuturn node if node will import name, or node
will import * from package. None is returned otherwise.
See test cases for examples. | _is_import_binding | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_util.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_util.py | MIT |
def tokenize_wrapper(input):
"""Tokenizes a string suppressing significant whitespace."""
skip = {token.NEWLINE, token.INDENT, token.DEDENT}
tokens = tokenize.generate_tokens(io.StringIO(input).readline)
for quintuple in tokens:
type, value, start, end, line_text = quintuple
if type not in skip:
yield quintuple | Tokenizes a string suppressing significant whitespace. | tokenize_wrapper | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/patcomp.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/patcomp.py | MIT |
def __init__(self, grammar_file=None):
"""Initializer.
Takes an optional alternative filename for the pattern grammar.
"""
if grammar_file is None:
self.grammar = pygram.pattern_grammar
self.syms = pygram.pattern_symbols
else:
self.grammar = driver.load_grammar(grammar_file)
self.syms = pygram.Symbols(self.grammar)
self.pygrammar = pygram.python_grammar
self.pysyms = pygram.python_symbols
self.driver = driver.Driver(self.grammar, convert=pattern_convert) | Initializer.
Takes an optional alternative filename for the pattern grammar. | __init__ | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/patcomp.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/patcomp.py | MIT |
def compile_pattern(self, input, debug=False, with_tree=False):
"""Compiles a pattern string to a nested pytree.*Pattern object."""
tokens = tokenize_wrapper(input)
try:
root = self.driver.parse_tokens(tokens, debug=debug)
except parse.ParseError as e:
raise PatternSyntaxError(str(e)) from None
if with_tree:
return self.compile_node(root), root
else:
return self.compile_node(root) | Compiles a pattern string to a nested pytree.*Pattern object. | compile_pattern | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/patcomp.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/patcomp.py | MIT |
def compile_node(self, node):
"""Compiles a node, recursively.
This is one big switch on the node type.
"""
# XXX Optimize certain Wildcard-containing-Wildcard patterns
# that can be merged
if node.type == self.syms.Matcher:
node = node.children[0] # Avoid unneeded recursion
if node.type == self.syms.Alternatives:
# Skip the odd children since they are just '|' tokens
alts = [self.compile_node(ch) for ch in node.children[::2]]
if len(alts) == 1:
return alts[0]
p = pytree.WildcardPattern([[a] for a in alts], min=1, max=1)
return p.optimize()
if node.type == self.syms.Alternative:
units = [self.compile_node(ch) for ch in node.children]
if len(units) == 1:
return units[0]
p = pytree.WildcardPattern([units], min=1, max=1)
return p.optimize()
if node.type == self.syms.NegatedUnit:
pattern = self.compile_basic(node.children[1:])
p = pytree.NegatedPattern(pattern)
return p.optimize()
assert node.type == self.syms.Unit
name = None
nodes = node.children
if len(nodes) >= 3 and nodes[1].type == token.EQUAL:
name = nodes[0].value
nodes = nodes[2:]
repeat = None
if len(nodes) >= 2 and nodes[-1].type == self.syms.Repeater:
repeat = nodes[-1]
nodes = nodes[:-1]
# Now we've reduced it to: STRING | NAME [Details] | (...) | [...]
pattern = self.compile_basic(nodes, repeat)
if repeat is not None:
assert repeat.type == self.syms.Repeater
children = repeat.children
child = children[0]
if child.type == token.STAR:
min = 0
max = pytree.HUGE
elif child.type == token.PLUS:
min = 1
max = pytree.HUGE
elif child.type == token.LBRACE:
assert children[-1].type == token.RBRACE
assert len(children) in (3, 5)
min = max = self.get_int(children[1])
if len(children) == 5:
max = self.get_int(children[3])
else:
assert False
if min != 1 or max != 1:
pattern = pattern.optimize()
pattern = pytree.WildcardPattern([[pattern]], min=min, max=max)
if name is not None:
pattern.name = name
return pattern.optimize() | Compiles a node, recursively.
This is one big switch on the node type. | compile_node | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/patcomp.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/patcomp.py | MIT |
def pattern_convert(grammar, raw_node_info):
"""Converts raw node information to a Node or Leaf instance."""
type, value, context, children = raw_node_info
if children or type in grammar.number2symbol:
return pytree.Node(type, children, context=context)
else:
return pytree.Leaf(type, value, context=context) | Converts raw node information to a Node or Leaf instance. | pattern_convert | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/patcomp.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/patcomp.py | MIT |
def diff_texts(a, b, filename):
"""Return a unified diff of two strings."""
a = a.splitlines()
b = b.splitlines()
return difflib.unified_diff(a, b, filename, filename,
"(original)", "(refactored)",
lineterm="") | Return a unified diff of two strings. | diff_texts | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/main.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/main.py | MIT |
def __init__(self, fixers, options, explicit, nobackups, show_diffs,
input_base_dir='', output_dir='', append_suffix=''):
"""
Args:
fixers: A list of fixers to import.
options: A dict with RefactoringTool configuration.
explicit: A list of fixers to run even if they are explicit.
nobackups: If true no backup '.bak' files will be created for those
files that are being refactored.
show_diffs: Should diffs of the refactoring be printed to stdout?
input_base_dir: The base directory for all input files. This class
will strip this path prefix off of filenames before substituting
it with output_dir. Only meaningful if output_dir is supplied.
All files processed by refactor() must start with this path.
output_dir: If supplied, all converted files will be written into
this directory tree instead of input_base_dir.
append_suffix: If supplied, all files output by this tool will have
this appended to their filename. Useful for changing .py to
.py3 for example by passing append_suffix='3'.
"""
self.nobackups = nobackups
self.show_diffs = show_diffs
if input_base_dir and not input_base_dir.endswith(os.sep):
input_base_dir += os.sep
self._input_base_dir = input_base_dir
self._output_dir = output_dir
self._append_suffix = append_suffix
super(StdoutRefactoringTool, self).__init__(fixers, options, explicit) | Args:
fixers: A list of fixers to import.
options: A dict with RefactoringTool configuration.
explicit: A list of fixers to run even if they are explicit.
nobackups: If true no backup '.bak' files will be created for those
files that are being refactored.
show_diffs: Should diffs of the refactoring be printed to stdout?
input_base_dir: The base directory for all input files. This class
will strip this path prefix off of filenames before substituting
it with output_dir. Only meaningful if output_dir is supplied.
All files processed by refactor() must start with this path.
output_dir: If supplied, all converted files will be written into
this directory tree instead of input_base_dir.
append_suffix: If supplied, all files output by this tool will have
this appended to their filename. Useful for changing .py to
.py3 for example by passing append_suffix='3'. | __init__ | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/main.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/main.py | MIT |
def main(fixer_pkg, args=None):
"""Main program.
Args:
fixer_pkg: the name of a package where the fixers are located.
args: optional; a list of command line arguments. If omitted,
sys.argv[1:] is used.
Returns a suggested exit status (0, 1, 2).
"""
# Set up option parser
parser = optparse.OptionParser(usage="2to3 [options] file|dir ...")
parser.add_option("-d", "--doctests_only", action="store_true",
help="Fix up doctests only")
parser.add_option("-f", "--fix", action="append", default=[],
help="Each FIX specifies a transformation; default: all")
parser.add_option("-j", "--processes", action="store", default=1,
type="int", help="Run 2to3 concurrently")
parser.add_option("-x", "--nofix", action="append", default=[],
help="Prevent a transformation from being run")
parser.add_option("-l", "--list-fixes", action="store_true",
help="List available transformations")
parser.add_option("-p", "--print-function", action="store_true",
help="Modify the grammar so that print() is a function")
parser.add_option("-v", "--verbose", action="store_true",
help="More verbose logging")
parser.add_option("--no-diffs", action="store_true",
help="Don't show diffs of the refactoring")
parser.add_option("-w", "--write", action="store_true",
help="Write back modified files")
parser.add_option("-n", "--nobackups", action="store_true", default=False,
help="Don't write backups for modified files")
parser.add_option("-o", "--output-dir", action="store", type="str",
default="", help="Put output files in this directory "
"instead of overwriting the input files. Requires -n.")
parser.add_option("-W", "--write-unchanged-files", action="store_true",
help="Also write files even if no changes were required"
" (useful with --output-dir); implies -w.")
parser.add_option("--add-suffix", action="store", type="str", default="",
help="Append this string to all output filenames."
" Requires -n if non-empty. "
"ex: --add-suffix='3' will generate .py3 files.")
# Parse command line arguments
refactor_stdin = False
flags = {}
options, args = parser.parse_args(args)
if options.write_unchanged_files:
flags["write_unchanged_files"] = True
if not options.write:
warn("--write-unchanged-files/-W implies -w.")
options.write = True
# If we allowed these, the original files would be renamed to backup names
# but not replaced.
if options.output_dir and not options.nobackups:
parser.error("Can't use --output-dir/-o without -n.")
if options.add_suffix and not options.nobackups:
parser.error("Can't use --add-suffix without -n.")
if not options.write and options.no_diffs:
warn("not writing files and not printing diffs; that's not very useful")
if not options.write and options.nobackups:
parser.error("Can't use -n without -w")
if options.list_fixes:
print("Available transformations for the -f/--fix option:")
for fixname in refactor.get_all_fix_names(fixer_pkg):
print(fixname)
if not args:
return 0
if not args:
print("At least one file or directory argument required.", file=sys.stderr)
print("Use --help to show usage.", file=sys.stderr)
return 2
if "-" in args:
refactor_stdin = True
if options.write:
print("Can't write to stdin.", file=sys.stderr)
return 2
if options.print_function:
flags["print_function"] = True
# Set up logging handler
level = logging.DEBUG if options.verbose else logging.INFO
logging.basicConfig(format='%(name)s: %(message)s', level=level)
logger = logging.getLogger('lib2to3.main')
# Initialize the refactoring tool
avail_fixes = set(refactor.get_fixers_from_package(fixer_pkg))
unwanted_fixes = set(fixer_pkg + ".fix_" + fix for fix in options.nofix)
explicit = set()
if options.fix:
all_present = False
for fix in options.fix:
if fix == "all":
all_present = True
else:
explicit.add(fixer_pkg + ".fix_" + fix)
requested = avail_fixes.union(explicit) if all_present else explicit
else:
requested = avail_fixes.union(explicit)
fixer_names = requested.difference(unwanted_fixes)
input_base_dir = os.path.commonprefix(args)
if (input_base_dir and not input_base_dir.endswith(os.sep)
and not os.path.isdir(input_base_dir)):
# One or more similar names were passed, their directory is the base.
# os.path.commonprefix() is ignorant of path elements, this corrects
# for that weird API.
input_base_dir = os.path.dirname(input_base_dir)
if options.output_dir:
input_base_dir = input_base_dir.rstrip(os.sep)
logger.info('Output in %r will mirror the input directory %r layout.',
options.output_dir, input_base_dir)
rt = StdoutRefactoringTool(
sorted(fixer_names), flags, sorted(explicit),
options.nobackups, not options.no_diffs,
input_base_dir=input_base_dir,
output_dir=options.output_dir,
append_suffix=options.add_suffix)
# Refactor all files and directories passed as arguments
if not rt.errors:
if refactor_stdin:
rt.refactor_stdin()
else:
try:
rt.refactor(args, options.write, options.doctests_only,
options.processes)
except refactor.MultiprocessingUnsupported:
assert options.processes > 1
print("Sorry, -j isn't supported on this platform.",
file=sys.stderr)
return 1
rt.summarize()
# Return error status (0 if rt.errors is zero)
return int(bool(rt.errors)) | Main program.
Args:
fixer_pkg: the name of a package where the fixers are located.
args: optional; a list of command line arguments. If omitted,
sys.argv[1:] is used.
Returns a suggested exit status (0, 1, 2). | main | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/main.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/main.py | MIT |
def __init__(self, options, log):
"""Initializer. Subclass may override.
Args:
options: a dict containing the options passed to RefactoringTool
that could be used to customize the fixer through the command line.
log: a list to append warnings and other messages to.
"""
self.options = options
self.log = log
self.compile_pattern() | Initializer. Subclass may override.
Args:
options: a dict containing the options passed to RefactoringTool
that could be used to customize the fixer through the command line.
log: a list to append warnings and other messages to. | __init__ | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_base.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_base.py | MIT |
def compile_pattern(self):
"""Compiles self.PATTERN into self.pattern.
Subclass may override if it doesn't want to use
self.{pattern,PATTERN} in .match().
"""
if self.PATTERN is not None:
PC = PatternCompiler()
self.pattern, self.pattern_tree = PC.compile_pattern(self.PATTERN,
with_tree=True) | Compiles self.PATTERN into self.pattern.
Subclass may override if it doesn't want to use
self.{pattern,PATTERN} in .match(). | compile_pattern | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_base.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_base.py | MIT |
def set_filename(self, filename):
"""Set the filename.
The main refactoring tool should call this.
"""
self.filename = filename | Set the filename.
The main refactoring tool should call this. | set_filename | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_base.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_base.py | MIT |
def match(self, node):
"""Returns match for a given parse tree node.
Should return a true or false object (not necessarily a bool).
It may return a non-empty dict of matching sub-nodes as
returned by a matching pattern.
Subclass may override.
"""
results = {"node": node}
return self.pattern.match(node, results) and results | Returns match for a given parse tree node.
Should return a true or false object (not necessarily a bool).
It may return a non-empty dict of matching sub-nodes as
returned by a matching pattern.
Subclass may override. | match | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_base.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_base.py | MIT |
def transform(self, node, results):
"""Returns the transformation for a given parse tree node.
Args:
node: the root of the parse tree that matched the fixer.
results: a dict mapping symbolic names to part of the match.
Returns:
None, or a node that is a modified copy of the
argument node. The node argument may also be modified in-place to
effect the same change.
Subclass *must* override.
"""
raise NotImplementedError() | Returns the transformation for a given parse tree node.
Args:
node: the root of the parse tree that matched the fixer.
results: a dict mapping symbolic names to part of the match.
Returns:
None, or a node that is a modified copy of the
argument node. The node argument may also be modified in-place to
effect the same change.
Subclass *must* override. | transform | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_base.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_base.py | MIT |
def new_name(self, template="xxx_todo_changeme"):
"""Return a string suitable for use as an identifier
The new name is guaranteed not to conflict with other identifiers.
"""
name = template
while name in self.used_names:
name = template + str(next(self.numbers))
self.used_names.add(name)
return name | Return a string suitable for use as an identifier
The new name is guaranteed not to conflict with other identifiers. | new_name | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_base.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_base.py | MIT |
def cannot_convert(self, node, reason=None):
"""Warn the user that a given chunk of code is not valid Python 3,
but that it cannot be converted automatically.
First argument is the top-level node for the code in question.
Optional second argument is why it can't be converted.
"""
lineno = node.get_lineno()
for_output = node.clone()
for_output.prefix = ""
msg = "Line %d: could not convert: %s"
self.log_message(msg % (lineno, for_output))
if reason:
self.log_message(reason) | Warn the user that a given chunk of code is not valid Python 3,
but that it cannot be converted automatically.
First argument is the top-level node for the code in question.
Optional second argument is why it can't be converted. | cannot_convert | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_base.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_base.py | MIT |
def warning(self, node, reason):
"""Used for warning the user about possible uncertainty in the
translation.
First argument is the top-level node for the code in question.
Optional second argument is why it can't be converted.
"""
lineno = node.get_lineno()
self.log_message("Line %d: %s" % (lineno, reason)) | Used for warning the user about possible uncertainty in the
translation.
First argument is the top-level node for the code in question.
Optional second argument is why it can't be converted. | warning | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_base.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_base.py | MIT |
def start_tree(self, tree, filename):
"""Some fixers need to maintain tree-wide state.
This method is called once, at the start of tree fix-up.
tree - the root node of the tree to be processed.
filename - the name of the file the tree came from.
"""
self.used_names = tree.used_names
self.set_filename(filename)
self.numbers = itertools.count(1)
self.first_log = True | Some fixers need to maintain tree-wide state.
This method is called once, at the start of tree fix-up.
tree - the root node of the tree to be processed.
filename - the name of the file the tree came from. | start_tree | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_base.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_base.py | MIT |
def finish_tree(self, tree, filename):
"""Some fixers need to maintain tree-wide state.
This method is called once, at the conclusion of tree fix-up.
tree - the root node of the tree to be processed.
filename - the name of the file the tree came from.
"""
pass | Some fixers need to maintain tree-wide state.
This method is called once, at the conclusion of tree fix-up.
tree - the root node of the tree to be processed.
filename - the name of the file the tree came from. | finish_tree | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_base.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/fixer_base.py | MIT |
def get_all_fix_names(fixer_pkg, remove_prefix=True):
"""Return a sorted list of all available fix names in the given package."""
pkg = __import__(fixer_pkg, [], [], ["*"])
fix_names = []
for finder, name, ispkg in pkgutil.iter_modules(pkg.__path__):
if name.startswith("fix_"):
if remove_prefix:
name = name[4:]
fix_names.append(name)
return fix_names | Return a sorted list of all available fix names in the given package. | get_all_fix_names | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | MIT |
def _get_head_types(pat):
""" Accepts a pytree Pattern Node and returns a set
of the pattern types which will match first. """
if isinstance(pat, (pytree.NodePattern, pytree.LeafPattern)):
# NodePatters must either have no type and no content
# or a type and content -- so they don't get any farther
# Always return leafs
if pat.type is None:
raise _EveryNode
return {pat.type}
if isinstance(pat, pytree.NegatedPattern):
if pat.content:
return _get_head_types(pat.content)
raise _EveryNode # Negated Patterns don't have a type
if isinstance(pat, pytree.WildcardPattern):
# Recurse on each node in content
r = set()
for p in pat.content:
for x in p:
r.update(_get_head_types(x))
return r
raise Exception("Oh no! I don't understand pattern %s" %(pat)) | Accepts a pytree Pattern Node and returns a set
of the pattern types which will match first. | _get_head_types | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | MIT |
def _get_headnode_dict(fixer_list):
""" Accepts a list of fixers and returns a dictionary
of head node type --> fixer list. """
head_nodes = collections.defaultdict(list)
every = []
for fixer in fixer_list:
if fixer.pattern:
try:
heads = _get_head_types(fixer.pattern)
except _EveryNode:
every.append(fixer)
else:
for node_type in heads:
head_nodes[node_type].append(fixer)
else:
if fixer._accept_type is not None:
head_nodes[fixer._accept_type].append(fixer)
else:
every.append(fixer)
for node_type in chain(pygram.python_grammar.symbol2number.values(),
pygram.python_grammar.tokens):
head_nodes[node_type].extend(every)
return dict(head_nodes) | Accepts a list of fixers and returns a dictionary
of head node type --> fixer list. | _get_headnode_dict | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | MIT |
def get_fixers_from_package(pkg_name):
"""
Return the fully qualified names for fixers in the package pkg_name.
"""
return [pkg_name + "." + fix_name
for fix_name in get_all_fix_names(pkg_name, False)] | Return the fully qualified names for fixers in the package pkg_name. | get_fixers_from_package | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | MIT |
def __init__(self, fixer_names, options=None, explicit=None):
"""Initializer.
Args:
fixer_names: a list of fixers to import
options: a dict with configuration.
explicit: a list of fixers to run even if they are explicit.
"""
self.fixers = fixer_names
self.explicit = explicit or []
self.options = self._default_options.copy()
if options is not None:
self.options.update(options)
if self.options["print_function"]:
self.grammar = pygram.python_grammar_no_print_statement
else:
self.grammar = pygram.python_grammar
# When this is True, the refactor*() methods will call write_file() for
# files processed even if they were not changed during refactoring. If
# and only if the refactor method's write parameter was True.
self.write_unchanged_files = self.options.get("write_unchanged_files")
self.errors = []
self.logger = logging.getLogger("RefactoringTool")
self.fixer_log = []
self.wrote = False
self.driver = driver.Driver(self.grammar,
convert=pytree.convert,
logger=self.logger)
self.pre_order, self.post_order = self.get_fixers()
self.files = [] # List of files that were or should be modified
self.BM = bm.BottomMatcher()
self.bmi_pre_order = [] # Bottom Matcher incompatible fixers
self.bmi_post_order = []
for fixer in chain(self.post_order, self.pre_order):
if fixer.BM_compatible:
self.BM.add_fixer(fixer)
# remove fixers that will be handled by the bottom-up
# matcher
elif fixer in self.pre_order:
self.bmi_pre_order.append(fixer)
elif fixer in self.post_order:
self.bmi_post_order.append(fixer)
self.bmi_pre_order_heads = _get_headnode_dict(self.bmi_pre_order)
self.bmi_post_order_heads = _get_headnode_dict(self.bmi_post_order) | Initializer.
Args:
fixer_names: a list of fixers to import
options: a dict with configuration.
explicit: a list of fixers to run even if they are explicit. | __init__ | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | MIT |
def get_fixers(self):
"""Inspects the options to load the requested patterns and handlers.
Returns:
(pre_order, post_order), where pre_order is the list of fixers that
want a pre-order AST traversal, and post_order is the list that want
post-order traversal.
"""
pre_order_fixers = []
post_order_fixers = []
for fix_mod_path in self.fixers:
mod = __import__(fix_mod_path, {}, {}, ["*"])
fix_name = fix_mod_path.rsplit(".", 1)[-1]
if fix_name.startswith(self.FILE_PREFIX):
fix_name = fix_name[len(self.FILE_PREFIX):]
parts = fix_name.split("_")
class_name = self.CLASS_PREFIX + "".join([p.title() for p in parts])
try:
fix_class = getattr(mod, class_name)
except AttributeError:
raise FixerError("Can't find %s.%s" % (fix_name, class_name)) from None
fixer = fix_class(self.options, self.fixer_log)
if fixer.explicit and self.explicit is not True and \
fix_mod_path not in self.explicit:
self.log_message("Skipping optional fixer: %s", fix_name)
continue
self.log_debug("Adding transformation: %s", fix_name)
if fixer.order == "pre":
pre_order_fixers.append(fixer)
elif fixer.order == "post":
post_order_fixers.append(fixer)
else:
raise FixerError("Illegal fixer order: %r" % fixer.order)
key_func = operator.attrgetter("run_order")
pre_order_fixers.sort(key=key_func)
post_order_fixers.sort(key=key_func)
return (pre_order_fixers, post_order_fixers) | Inspects the options to load the requested patterns and handlers.
Returns:
(pre_order, post_order), where pre_order is the list of fixers that
want a pre-order AST traversal, and post_order is the list that want
post-order traversal. | get_fixers | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | MIT |
def log_error(self, msg, *args, **kwds):
"""Called when an error occurs."""
raise | Called when an error occurs. | log_error | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | MIT |
def log_message(self, msg, *args):
"""Hook to log a message."""
if args:
msg = msg % args
self.logger.info(msg) | Hook to log a message. | log_message | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | MIT |
def print_output(self, old_text, new_text, filename, equal):
"""Called with the old version, new version, and filename of a
refactored file."""
pass | Called with the old version, new version, and filename of a
refactored file. | print_output | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | MIT |
def refactor(self, items, write=False, doctests_only=False):
"""Refactor a list of files and directories."""
for dir_or_file in items:
if os.path.isdir(dir_or_file):
self.refactor_dir(dir_or_file, write, doctests_only)
else:
self.refactor_file(dir_or_file, write, doctests_only) | Refactor a list of files and directories. | refactor | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | MIT |
def refactor_dir(self, dir_name, write=False, doctests_only=False):
"""Descends down a directory and refactor every Python file found.
Python files are assumed to have a .py extension.
Files and subdirectories starting with '.' are skipped.
"""
py_ext = os.extsep + "py"
for dirpath, dirnames, filenames in os.walk(dir_name):
self.log_debug("Descending into %s", dirpath)
dirnames.sort()
filenames.sort()
for name in filenames:
if (not name.startswith(".") and
os.path.splitext(name)[1] == py_ext):
fullname = os.path.join(dirpath, name)
self.refactor_file(fullname, write, doctests_only)
# Modify dirnames in-place to remove subdirs with leading dots
dirnames[:] = [dn for dn in dirnames if not dn.startswith(".")] | Descends down a directory and refactor every Python file found.
Python files are assumed to have a .py extension.
Files and subdirectories starting with '.' are skipped. | refactor_dir | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | MIT |
def _read_python_source(self, filename):
"""
Do our best to decode a Python source file correctly.
"""
try:
f = open(filename, "rb")
except OSError as err:
self.log_error("Can't open %s: %s", filename, err)
return None, None
try:
encoding = tokenize.detect_encoding(f.readline)[0]
finally:
f.close()
with io.open(filename, "r", encoding=encoding, newline='') as f:
return f.read(), encoding | Do our best to decode a Python source file correctly. | _read_python_source | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | MIT |
def refactor_file(self, filename, write=False, doctests_only=False):
"""Refactors a file."""
input, encoding = self._read_python_source(filename)
if input is None:
# Reading the file failed.
return
input += "\n" # Silence certain parse errors
if doctests_only:
self.log_debug("Refactoring doctests in %s", filename)
output = self.refactor_docstring(input, filename)
if self.write_unchanged_files or output != input:
self.processed_file(output, filename, input, write, encoding)
else:
self.log_debug("No doctest changes in %s", filename)
else:
tree = self.refactor_string(input, filename)
if self.write_unchanged_files or (tree and tree.was_changed):
# The [:-1] is to take off the \n we added earlier
self.processed_file(str(tree)[:-1], filename,
write=write, encoding=encoding)
else:
self.log_debug("No changes in %s", filename) | Refactors a file. | refactor_file | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | MIT |
def refactor_string(self, data, name):
"""Refactor a given input string.
Args:
data: a string holding the code to be refactored.
name: a human-readable name for use in error/log messages.
Returns:
An AST corresponding to the refactored input stream; None if
there were errors during the parse.
"""
features = _detect_future_features(data)
if "print_function" in features:
self.driver.grammar = pygram.python_grammar_no_print_statement
try:
tree = self.driver.parse_string(data)
except Exception as err:
self.log_error("Can't parse %s: %s: %s",
name, err.__class__.__name__, err)
return
finally:
self.driver.grammar = self.grammar
tree.future_features = features
self.log_debug("Refactoring %s", name)
self.refactor_tree(tree, name)
return tree | Refactor a given input string.
Args:
data: a string holding the code to be refactored.
name: a human-readable name for use in error/log messages.
Returns:
An AST corresponding to the refactored input stream; None if
there were errors during the parse. | refactor_string | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | MIT |
def refactor_tree(self, tree, name):
"""Refactors a parse tree (modifying the tree in place).
For compatible patterns the bottom matcher module is
used. Otherwise the tree is traversed node-to-node for
matches.
Args:
tree: a pytree.Node instance representing the root of the tree
to be refactored.
name: a human-readable name for this tree.
Returns:
True if the tree was modified, False otherwise.
"""
for fixer in chain(self.pre_order, self.post_order):
fixer.start_tree(tree, name)
#use traditional matching for the incompatible fixers
self.traverse_by(self.bmi_pre_order_heads, tree.pre_order())
self.traverse_by(self.bmi_post_order_heads, tree.post_order())
# obtain a set of candidate nodes
match_set = self.BM.run(tree.leaves())
while any(match_set.values()):
for fixer in self.BM.fixers:
if fixer in match_set and match_set[fixer]:
#sort by depth; apply fixers from bottom(of the AST) to top
match_set[fixer].sort(key=pytree.Base.depth, reverse=True)
if fixer.keep_line_order:
#some fixers(eg fix_imports) must be applied
#with the original file's line order
match_set[fixer].sort(key=pytree.Base.get_lineno)
for node in list(match_set[fixer]):
if node in match_set[fixer]:
match_set[fixer].remove(node)
try:
find_root(node)
except ValueError:
# this node has been cut off from a
# previous transformation ; skip
continue
if node.fixers_applied and fixer in node.fixers_applied:
# do not apply the same fixer again
continue
results = fixer.match(node)
if results:
new = fixer.transform(node, results)
if new is not None:
node.replace(new)
#new.fixers_applied.append(fixer)
for node in new.post_order():
# do not apply the fixer again to
# this or any subnode
if not node.fixers_applied:
node.fixers_applied = []
node.fixers_applied.append(fixer)
# update the original match set for
# the added code
new_matches = self.BM.run(new.leaves())
for fxr in new_matches:
if not fxr in match_set:
match_set[fxr]=[]
match_set[fxr].extend(new_matches[fxr])
for fixer in chain(self.pre_order, self.post_order):
fixer.finish_tree(tree, name)
return tree.was_changed | Refactors a parse tree (modifying the tree in place).
For compatible patterns the bottom matcher module is
used. Otherwise the tree is traversed node-to-node for
matches.
Args:
tree: a pytree.Node instance representing the root of the tree
to be refactored.
name: a human-readable name for this tree.
Returns:
True if the tree was modified, False otherwise. | refactor_tree | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | MIT |
def traverse_by(self, fixers, traversal):
"""Traverse an AST, applying a set of fixers to each node.
This is a helper method for refactor_tree().
Args:
fixers: a list of fixer instances.
traversal: a generator that yields AST nodes.
Returns:
None
"""
if not fixers:
return
for node in traversal:
for fixer in fixers[node.type]:
results = fixer.match(node)
if results:
new = fixer.transform(node, results)
if new is not None:
node.replace(new)
node = new | Traverse an AST, applying a set of fixers to each node.
This is a helper method for refactor_tree().
Args:
fixers: a list of fixer instances.
traversal: a generator that yields AST nodes.
Returns:
None | traverse_by | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | MIT |
def processed_file(self, new_text, filename, old_text=None, write=False,
encoding=None):
"""
Called when a file has been refactored and there may be changes.
"""
self.files.append(filename)
if old_text is None:
old_text = self._read_python_source(filename)[0]
if old_text is None:
return
equal = old_text == new_text
self.print_output(old_text, new_text, filename, equal)
if equal:
self.log_debug("No changes to %s", filename)
if not self.write_unchanged_files:
return
if write:
self.write_file(new_text, filename, old_text, encoding)
else:
self.log_debug("Not writing changes to %s", filename) | Called when a file has been refactored and there may be changes. | processed_file | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | MIT |
def write_file(self, new_text, filename, old_text, encoding=None):
"""Writes a string to a file.
It first shows a unified diff between the old text and the new text, and
then rewrites the file; the latter is only done if the write option is
set.
"""
try:
fp = io.open(filename, "w", encoding=encoding, newline='')
except OSError as err:
self.log_error("Can't create %s: %s", filename, err)
return
with fp:
try:
fp.write(new_text)
except OSError as err:
self.log_error("Can't write %s: %s", filename, err)
self.log_debug("Wrote changes to %s", filename)
self.wrote = True | Writes a string to a file.
It first shows a unified diff between the old text and the new text, and
then rewrites the file; the latter is only done if the write option is
set. | write_file | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | MIT |
def refactor_docstring(self, input, filename):
"""Refactors a docstring, looking for doctests.
This returns a modified version of the input string. It looks
for doctests, which start with a ">>>" prompt, and may be
continued with "..." prompts, as long as the "..." is indented
the same as the ">>>".
(Unfortunately we can't use the doctest module's parser,
since, like most parsers, it is not geared towards preserving
the original source.)
"""
result = []
block = None
block_lineno = None
indent = None
lineno = 0
for line in input.splitlines(keepends=True):
lineno += 1
if line.lstrip().startswith(self.PS1):
if block is not None:
result.extend(self.refactor_doctest(block, block_lineno,
indent, filename))
block_lineno = lineno
block = [line]
i = line.find(self.PS1)
indent = line[:i]
elif (indent is not None and
(line.startswith(indent + self.PS2) or
line == indent + self.PS2.rstrip() + "\n")):
block.append(line)
else:
if block is not None:
result.extend(self.refactor_doctest(block, block_lineno,
indent, filename))
block = None
indent = None
result.append(line)
if block is not None:
result.extend(self.refactor_doctest(block, block_lineno,
indent, filename))
return "".join(result) | Refactors a docstring, looking for doctests.
This returns a modified version of the input string. It looks
for doctests, which start with a ">>>" prompt, and may be
continued with "..." prompts, as long as the "..." is indented
the same as the ">>>".
(Unfortunately we can't use the doctest module's parser,
since, like most parsers, it is not geared towards preserving
the original source.) | refactor_docstring | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | MIT |
def refactor_doctest(self, block, lineno, indent, filename):
"""Refactors one doctest.
A doctest is given as a block of lines, the first of which starts
with ">>>" (possibly indented), while the remaining lines start
with "..." (identically indented).
"""
try:
tree = self.parse_block(block, lineno, indent)
except Exception as err:
if self.logger.isEnabledFor(logging.DEBUG):
for line in block:
self.log_debug("Source: %s", line.rstrip("\n"))
self.log_error("Can't parse docstring in %s line %s: %s: %s",
filename, lineno, err.__class__.__name__, err)
return block
if self.refactor_tree(tree, filename):
new = str(tree).splitlines(keepends=True)
# Undo the adjustment of the line numbers in wrap_toks() below.
clipped, new = new[:lineno-1], new[lineno-1:]
assert clipped == ["\n"] * (lineno-1), clipped
if not new[-1].endswith("\n"):
new[-1] += "\n"
block = [indent + self.PS1 + new.pop(0)]
if new:
block += [indent + self.PS2 + line for line in new]
return block | Refactors one doctest.
A doctest is given as a block of lines, the first of which starts
with ">>>" (possibly indented), while the remaining lines start
with "..." (identically indented). | refactor_doctest | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | MIT |
def parse_block(self, block, lineno, indent):
"""Parses a block into a tree.
This is necessary to get correct line number / offset information
in the parser diagnostics and embedded into the parse tree.
"""
tree = self.driver.parse_tokens(self.wrap_toks(block, lineno, indent))
tree.future_features = frozenset()
return tree | Parses a block into a tree.
This is necessary to get correct line number / offset information
in the parser diagnostics and embedded into the parse tree. | parse_block | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | MIT |
def wrap_toks(self, block, lineno, indent):
"""Wraps a tokenize stream to systematically modify start/end."""
tokens = tokenize.generate_tokens(self.gen_lines(block, indent).__next__)
for type, value, (line0, col0), (line1, col1), line_text in tokens:
line0 += lineno - 1
line1 += lineno - 1
# Don't bother updating the columns; this is too complicated
# since line_text would also have to be updated and it would
# still break for tokens spanning lines. Let the user guess
# that the column numbers for doctests are relative to the
# end of the prompt string (PS1 or PS2).
yield type, value, (line0, col0), (line1, col1), line_text | Wraps a tokenize stream to systematically modify start/end. | wrap_toks | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | MIT |
def gen_lines(self, block, indent):
"""Generates lines as expected by tokenize from a list of lines.
This strips the first len(indent + self.PS1) characters off each line.
"""
prefix1 = indent + self.PS1
prefix2 = indent + self.PS2
prefix = prefix1
for line in block:
if line.startswith(prefix):
yield line[len(prefix):]
elif line == prefix.rstrip() + "\n":
yield "\n"
else:
raise AssertionError("line=%r, prefix=%r" % (line, prefix))
prefix = prefix2
while True:
yield "" | Generates lines as expected by tokenize from a list of lines.
This strips the first len(indent + self.PS1) characters off each line. | gen_lines | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/refactor.py | MIT |
def leaf_to_root(self):
"""Internal method. Returns a characteristic path of the
pattern tree. This method must be run for all leaves until the
linear subpatterns are merged into a single"""
node = self
subp = []
while node:
if node.type == TYPE_ALTERNATIVES:
node.alternatives.append(subp)
if len(node.alternatives) == len(node.children):
#last alternative
subp = [tuple(node.alternatives)]
node.alternatives = []
node = node.parent
continue
else:
node = node.parent
subp = None
break
if node.type == TYPE_GROUP:
node.group.append(subp)
#probably should check the number of leaves
if len(node.group) == len(node.children):
subp = get_characteristic_subpattern(node.group)
node.group = []
node = node.parent
continue
else:
node = node.parent
subp = None
break
if node.type == token_labels.NAME and node.name:
#in case of type=name, use the name instead
subp.append(node.name)
else:
subp.append(node.type)
node = node.parent
return subp | Internal method. Returns a characteristic path of the
pattern tree. This method must be run for all leaves until the
linear subpatterns are merged into a single | leaf_to_root | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/btm_utils.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/btm_utils.py | MIT |
def get_linear_subpattern(self):
"""Drives the leaf_to_root method. The reason that
leaf_to_root must be run multiple times is because we need to
reject 'group' matches; for example the alternative form
(a | b c) creates a group [b c] that needs to be matched. Since
matching multiple linear patterns overcomes the automaton's
capabilities, leaf_to_root merges each group into a single
choice based on 'characteristic'ity,
i.e. (a|b c) -> (a|b) if b more characteristic than c
Returns: The most 'characteristic'(as defined by
get_characteristic_subpattern) path for the compiled pattern
tree.
"""
for l in self.leaves():
subp = l.leaf_to_root()
if subp:
return subp | Drives the leaf_to_root method. The reason that
leaf_to_root must be run multiple times is because we need to
reject 'group' matches; for example the alternative form
(a | b c) creates a group [b c] that needs to be matched. Since
matching multiple linear patterns overcomes the automaton's
capabilities, leaf_to_root merges each group into a single
choice based on 'characteristic'ity,
i.e. (a|b c) -> (a|b) if b more characteristic than c
Returns: The most 'characteristic'(as defined by
get_characteristic_subpattern) path for the compiled pattern
tree. | get_linear_subpattern | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/btm_utils.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/btm_utils.py | MIT |
def reduce_tree(node, parent=None):
"""
Internal function. Reduces a compiled pattern tree to an
intermediate representation suitable for feeding the
automaton. This also trims off any optional pattern elements(like
[a], a*).
"""
new_node = None
#switch on the node type
if node.type == syms.Matcher:
#skip
node = node.children[0]
if node.type == syms.Alternatives :
#2 cases
if len(node.children) <= 2:
#just a single 'Alternative', skip this node
new_node = reduce_tree(node.children[0], parent)
else:
#real alternatives
new_node = MinNode(type=TYPE_ALTERNATIVES)
#skip odd children('|' tokens)
for child in node.children:
if node.children.index(child)%2:
continue
reduced = reduce_tree(child, new_node)
if reduced is not None:
new_node.children.append(reduced)
elif node.type == syms.Alternative:
if len(node.children) > 1:
new_node = MinNode(type=TYPE_GROUP)
for child in node.children:
reduced = reduce_tree(child, new_node)
if reduced:
new_node.children.append(reduced)
if not new_node.children:
# delete the group if all of the children were reduced to None
new_node = None
else:
new_node = reduce_tree(node.children[0], parent)
elif node.type == syms.Unit:
if (isinstance(node.children[0], pytree.Leaf) and
node.children[0].value == '('):
#skip parentheses
return reduce_tree(node.children[1], parent)
if ((isinstance(node.children[0], pytree.Leaf) and
node.children[0].value == '[')
or
(len(node.children)>1 and
hasattr(node.children[1], "value") and
node.children[1].value == '[')):
#skip whole unit if its optional
return None
leaf = True
details_node = None
alternatives_node = None
has_repeater = False
repeater_node = None
has_variable_name = False
for child in node.children:
if child.type == syms.Details:
leaf = False
details_node = child
elif child.type == syms.Repeater:
has_repeater = True
repeater_node = child
elif child.type == syms.Alternatives:
alternatives_node = child
if hasattr(child, 'value') and child.value == '=': # variable name
has_variable_name = True
#skip variable name
if has_variable_name:
#skip variable name, '='
name_leaf = node.children[2]
if hasattr(name_leaf, 'value') and name_leaf.value == '(':
# skip parenthesis
name_leaf = node.children[3]
else:
name_leaf = node.children[0]
#set node type
if name_leaf.type == token_labels.NAME:
#(python) non-name or wildcard
if name_leaf.value == 'any':
new_node = MinNode(type=TYPE_ANY)
else:
if hasattr(token_labels, name_leaf.value):
new_node = MinNode(type=getattr(token_labels, name_leaf.value))
else:
new_node = MinNode(type=getattr(pysyms, name_leaf.value))
elif name_leaf.type == token_labels.STRING:
#(python) name or character; remove the apostrophes from
#the string value
name = name_leaf.value.strip("'")
if name in tokens:
new_node = MinNode(type=tokens[name])
else:
new_node = MinNode(type=token_labels.NAME, name=name)
elif name_leaf.type == syms.Alternatives:
new_node = reduce_tree(alternatives_node, parent)
#handle repeaters
if has_repeater:
if repeater_node.children[0].value == '*':
#reduce to None
new_node = None
elif repeater_node.children[0].value == '+':
#reduce to a single occurrence i.e. do nothing
pass
else:
#TODO: handle {min, max} repeaters
raise NotImplementedError
pass
#add children
if details_node and new_node is not None:
for child in details_node.children[1:-1]:
#skip '<', '>' markers
reduced = reduce_tree(child, new_node)
if reduced is not None:
new_node.children.append(reduced)
if new_node:
new_node.parent = parent
return new_node | Internal function. Reduces a compiled pattern tree to an
intermediate representation suitable for feeding the
automaton. This also trims off any optional pattern elements(like
[a], a*). | reduce_tree | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/btm_utils.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/btm_utils.py | MIT |
def get_characteristic_subpattern(subpatterns):
"""Picks the most characteristic from a list of linear patterns
Current order used is:
names > common_names > common_chars
"""
if not isinstance(subpatterns, list):
return subpatterns
if len(subpatterns)==1:
return subpatterns[0]
# first pick out the ones containing variable names
subpatterns_with_names = []
subpatterns_with_common_names = []
common_names = ['in', 'for', 'if' , 'not', 'None']
subpatterns_with_common_chars = []
common_chars = "[]().,:"
for subpattern in subpatterns:
if any(rec_test(subpattern, lambda x: type(x) is str)):
if any(rec_test(subpattern,
lambda x: isinstance(x, str) and x in common_chars)):
subpatterns_with_common_chars.append(subpattern)
elif any(rec_test(subpattern,
lambda x: isinstance(x, str) and x in common_names)):
subpatterns_with_common_names.append(subpattern)
else:
subpatterns_with_names.append(subpattern)
if subpatterns_with_names:
subpatterns = subpatterns_with_names
elif subpatterns_with_common_names:
subpatterns = subpatterns_with_common_names
elif subpatterns_with_common_chars:
subpatterns = subpatterns_with_common_chars
# of the remaining subpatterns pick out the longest one
return max(subpatterns, key=len) | Picks the most characteristic from a list of linear patterns
Current order used is:
names > common_names > common_chars | get_characteristic_subpattern | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/btm_utils.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/btm_utils.py | MIT |
def rec_test(sequence, test_func):
"""Tests test_func on all items of sequence and items of included
sub-iterables"""
for x in sequence:
if isinstance(x, (list, tuple)):
yield from rec_test(x, test_func)
else:
yield test_func(x) | Tests test_func on all items of sequence and items of included
sub-iterables | rec_test | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/btm_utils.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/btm_utils.py | MIT |
def __new__(cls, *args, **kwds):
"""Constructor that prevents Base from being instantiated."""
assert cls is not Base, "Cannot instantiate Base"
return object.__new__(cls) | Constructor that prevents Base from being instantiated. | __new__ | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def __eq__(self, other):
"""
Compare two nodes for equality.
This calls the method _eq().
"""
if self.__class__ is not other.__class__:
return NotImplemented
return self._eq(other) | Compare two nodes for equality.
This calls the method _eq(). | __eq__ | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def _eq(self, other):
"""
Compare two nodes for equality.
This is called by __eq__ and __ne__. It is only called if the two nodes
have the same type. This must be implemented by the concrete subclass.
Nodes should be considered equal if they have the same structure,
ignoring the prefix string and other context information.
"""
raise NotImplementedError | Compare two nodes for equality.
This is called by __eq__ and __ne__. It is only called if the two nodes
have the same type. This must be implemented by the concrete subclass.
Nodes should be considered equal if they have the same structure,
ignoring the prefix string and other context information. | _eq | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def clone(self):
"""
Return a cloned (deep) copy of self.
This must be implemented by the concrete subclass.
"""
raise NotImplementedError | Return a cloned (deep) copy of self.
This must be implemented by the concrete subclass. | clone | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def post_order(self):
"""
Return a post-order iterator for the tree.
This must be implemented by the concrete subclass.
"""
raise NotImplementedError | Return a post-order iterator for the tree.
This must be implemented by the concrete subclass. | post_order | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def pre_order(self):
"""
Return a pre-order iterator for the tree.
This must be implemented by the concrete subclass.
"""
raise NotImplementedError | Return a pre-order iterator for the tree.
This must be implemented by the concrete subclass. | pre_order | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def replace(self, new):
"""Replace this node with a new one in the parent."""
assert self.parent is not None, str(self)
assert new is not None
if not isinstance(new, list):
new = [new]
l_children = []
found = False
for ch in self.parent.children:
if ch is self:
assert not found, (self.parent.children, self, new)
if new is not None:
l_children.extend(new)
found = True
else:
l_children.append(ch)
assert found, (self.children, self, new)
self.parent.changed()
self.parent.children = l_children
for x in new:
x.parent = self.parent
self.parent = None | Replace this node with a new one in the parent. | replace | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def get_lineno(self):
"""Return the line number which generated the invocant node."""
node = self
while not isinstance(node, Leaf):
if not node.children:
return
node = node.children[0]
return node.lineno | Return the line number which generated the invocant node. | get_lineno | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def remove(self):
"""
Remove the node from the tree. Returns the position of the node in its
parent's children before it was removed.
"""
if self.parent:
for i, node in enumerate(self.parent.children):
if node is self:
self.parent.changed()
del self.parent.children[i]
self.parent = None
return i | Remove the node from the tree. Returns the position of the node in its
parent's children before it was removed. | remove | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def next_sibling(self):
"""
The node immediately following the invocant in their parent's children
list. If the invocant does not have a next sibling, it is None
"""
if self.parent is None:
return None
# Can't use index(); we need to test by identity
for i, child in enumerate(self.parent.children):
if child is self:
try:
return self.parent.children[i+1]
except IndexError:
return None | The node immediately following the invocant in their parent's children
list. If the invocant does not have a next sibling, it is None | next_sibling | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def prev_sibling(self):
"""
The node immediately preceding the invocant in their parent's children
list. If the invocant does not have a previous sibling, it is None.
"""
if self.parent is None:
return None
# Can't use index(); we need to test by identity
for i, child in enumerate(self.parent.children):
if child is self:
if i == 0:
return None
return self.parent.children[i-1] | The node immediately preceding the invocant in their parent's children
list. If the invocant does not have a previous sibling, it is None. | prev_sibling | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def get_suffix(self):
"""
Return the string immediately following the invocant node. This is
effectively equivalent to node.next_sibling.prefix
"""
next_sib = self.next_sibling
if next_sib is None:
return ""
return next_sib.prefix | Return the string immediately following the invocant node. This is
effectively equivalent to node.next_sibling.prefix | get_suffix | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def __init__(self,type, children,
context=None,
prefix=None,
fixers_applied=None):
"""
Initializer.
Takes a type constant (a symbol number >= 256), a sequence of
child nodes, and an optional context keyword argument.
As a side effect, the parent pointers of the children are updated.
"""
assert type >= 256, type
self.type = type
self.children = list(children)
for ch in self.children:
assert ch.parent is None, repr(ch)
ch.parent = self
if prefix is not None:
self.prefix = prefix
if fixers_applied:
self.fixers_applied = fixers_applied[:]
else:
self.fixers_applied = None | Initializer.
Takes a type constant (a symbol number >= 256), a sequence of
child nodes, and an optional context keyword argument.
As a side effect, the parent pointers of the children are updated. | __init__ | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def __repr__(self):
"""Return a canonical string representation."""
return "%s(%s, %r)" % (self.__class__.__name__,
type_repr(self.type),
self.children) | Return a canonical string representation. | __repr__ | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def __unicode__(self):
"""
Return a pretty string representation.
This reproduces the input source exactly.
"""
return "".join(map(str, self.children)) | Return a pretty string representation.
This reproduces the input source exactly. | __unicode__ | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def _eq(self, other):
"""Compare two nodes for equality."""
return (self.type, self.children) == (other.type, other.children) | Compare two nodes for equality. | _eq | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def clone(self):
"""Return a cloned (deep) copy of self."""
return Node(self.type, [ch.clone() for ch in self.children],
fixers_applied=self.fixers_applied) | Return a cloned (deep) copy of self. | clone | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def post_order(self):
"""Return a post-order iterator for the tree."""
for child in self.children:
yield from child.post_order()
yield self | Return a post-order iterator for the tree. | post_order | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def pre_order(self):
"""Return a pre-order iterator for the tree."""
yield self
for child in self.children:
yield from child.pre_order() | Return a pre-order iterator for the tree. | pre_order | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def prefix(self):
"""
The whitespace and comments preceding this node in the input.
"""
if not self.children:
return ""
return self.children[0].prefix | The whitespace and comments preceding this node in the input. | prefix | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def set_child(self, i, child):
"""
Equivalent to 'node.children[i] = child'. This method also sets the
child's parent attribute appropriately.
"""
child.parent = self
self.children[i].parent = None
self.children[i] = child
self.changed() | Equivalent to 'node.children[i] = child'. This method also sets the
child's parent attribute appropriately. | set_child | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def insert_child(self, i, child):
"""
Equivalent to 'node.children.insert(i, child)'. This method also sets
the child's parent attribute appropriately.
"""
child.parent = self
self.children.insert(i, child)
self.changed() | Equivalent to 'node.children.insert(i, child)'. This method also sets
the child's parent attribute appropriately. | insert_child | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def append_child(self, child):
"""
Equivalent to 'node.children.append(child)'. This method also sets the
child's parent attribute appropriately.
"""
child.parent = self
self.children.append(child)
self.changed() | Equivalent to 'node.children.append(child)'. This method also sets the
child's parent attribute appropriately. | append_child | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def __init__(self, type, value,
context=None,
prefix=None,
fixers_applied=[]):
"""
Initializer.
Takes a type constant (a token number < 256), a string value, and an
optional context keyword argument.
"""
assert 0 <= type < 256, type
if context is not None:
self._prefix, (self.lineno, self.column) = context
self.type = type
self.value = value
if prefix is not None:
self._prefix = prefix
self.fixers_applied = fixers_applied[:] | Initializer.
Takes a type constant (a token number < 256), a string value, and an
optional context keyword argument. | __init__ | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def __repr__(self):
"""Return a canonical string representation."""
return "%s(%r, %r)" % (self.__class__.__name__,
self.type,
self.value) | Return a canonical string representation. | __repr__ | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def __unicode__(self):
"""
Return a pretty string representation.
This reproduces the input source exactly.
"""
return self.prefix + str(self.value) | Return a pretty string representation.
This reproduces the input source exactly. | __unicode__ | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def _eq(self, other):
"""Compare two nodes for equality."""
return (self.type, self.value) == (other.type, other.value) | Compare two nodes for equality. | _eq | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def clone(self):
"""Return a cloned (deep) copy of self."""
return Leaf(self.type, self.value,
(self.prefix, (self.lineno, self.column)),
fixers_applied=self.fixers_applied) | Return a cloned (deep) copy of self. | clone | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def post_order(self):
"""Return a post-order iterator for the tree."""
yield self | Return a post-order iterator for the tree. | post_order | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def pre_order(self):
"""Return a pre-order iterator for the tree."""
yield self | Return a pre-order iterator for the tree. | pre_order | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def prefix(self):
"""
The whitespace and comments preceding this token in the input.
"""
return self._prefix | The whitespace and comments preceding this token in the input. | prefix | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def convert(gr, raw_node):
"""
Convert raw node information to a Node or Leaf instance.
This is passed to the parser driver which calls it whenever a reduction of a
grammar rule produces a new complete node, so that the tree is build
strictly bottom-up.
"""
type, value, context, children = raw_node
if children or type in gr.number2symbol:
# If there's exactly one child, return that child instead of
# creating a new node.
if len(children) == 1:
return children[0]
return Node(type, children, context=context)
else:
return Leaf(type, value, context=context) | Convert raw node information to a Node or Leaf instance.
This is passed to the parser driver which calls it whenever a reduction of a
grammar rule produces a new complete node, so that the tree is build
strictly bottom-up. | convert | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def __new__(cls, *args, **kwds):
"""Constructor that prevents BasePattern from being instantiated."""
assert cls is not BasePattern, "Cannot instantiate BasePattern"
return object.__new__(cls) | Constructor that prevents BasePattern from being instantiated. | __new__ | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def optimize(self):
"""
A subclass can define this as a hook for optimizations.
Returns either self or another node with the same effect.
"""
return self | A subclass can define this as a hook for optimizations.
Returns either self or another node with the same effect. | optimize | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def match(self, node, results=None):
"""
Does this pattern exactly match a node?
Returns True if it matches, False if not.
If results is not None, it must be a dict which will be
updated with the nodes matching named subpatterns.
Default implementation for non-wildcard patterns.
"""
if self.type is not None and node.type != self.type:
return False
if self.content is not None:
r = None
if results is not None:
r = {}
if not self._submatch(node, r):
return False
if r:
results.update(r)
if results is not None and self.name:
results[self.name] = node
return True | Does this pattern exactly match a node?
Returns True if it matches, False if not.
If results is not None, it must be a dict which will be
updated with the nodes matching named subpatterns.
Default implementation for non-wildcard patterns. | match | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def match_seq(self, nodes, results=None):
"""
Does this pattern exactly match a sequence of nodes?
Default implementation for non-wildcard patterns.
"""
if len(nodes) != 1:
return False
return self.match(nodes[0], results) | Does this pattern exactly match a sequence of nodes?
Default implementation for non-wildcard patterns. | match_seq | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def generate_matches(self, nodes):
"""
Generator yielding all matches for this pattern.
Default implementation for non-wildcard patterns.
"""
r = {}
if nodes and self.match(nodes[0], r):
yield 1, r | Generator yielding all matches for this pattern.
Default implementation for non-wildcard patterns. | generate_matches | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def __init__(self, type=None, content=None, name=None):
"""
Initializer. Takes optional type, content, and name.
The type, if given must be a token type (< 256). If not given,
this matches any *leaf* node; the content may still be required.
The content, if given, must be a string.
If a name is given, the matching node is stored in the results
dict under that key.
"""
if type is not None:
assert 0 <= type < 256, type
if content is not None:
assert isinstance(content, str), repr(content)
self.type = type
self.content = content
self.name = name | Initializer. Takes optional type, content, and name.
The type, if given must be a token type (< 256). If not given,
this matches any *leaf* node; the content may still be required.
The content, if given, must be a string.
If a name is given, the matching node is stored in the results
dict under that key. | __init__ | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def match(self, node, results=None):
"""Override match() to insist on a leaf node."""
if not isinstance(node, Leaf):
return False
return BasePattern.match(self, node, results) | Override match() to insist on a leaf node. | match | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def _submatch(self, node, results=None):
"""
Match the pattern's content to the node's children.
This assumes the node type matches and self.content is not None.
Returns True if it matches, False if not.
If results is not None, it must be a dict which will be
updated with the nodes matching named subpatterns.
When returning False, the results dict may still be updated.
"""
return self.content == node.value | Match the pattern's content to the node's children.
This assumes the node type matches and self.content is not None.
Returns True if it matches, False if not.
If results is not None, it must be a dict which will be
updated with the nodes matching named subpatterns.
When returning False, the results dict may still be updated. | _submatch | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def __init__(self, type=None, content=None, name=None):
"""
Initializer. Takes optional type, content, and name.
The type, if given, must be a symbol type (>= 256). If the
type is None this matches *any* single node (leaf or not),
except if content is not None, in which it only matches
non-leaf nodes that also match the content pattern.
The content, if not None, must be a sequence of Patterns that
must match the node's children exactly. If the content is
given, the type must not be None.
If a name is given, the matching node is stored in the results
dict under that key.
"""
if type is not None:
assert type >= 256, type
if content is not None:
assert not isinstance(content, str), repr(content)
content = list(content)
for i, item in enumerate(content):
assert isinstance(item, BasePattern), (i, item)
if isinstance(item, WildcardPattern):
self.wildcards = True
self.type = type
self.content = content
self.name = name | Initializer. Takes optional type, content, and name.
The type, if given, must be a symbol type (>= 256). If the
type is None this matches *any* single node (leaf or not),
except if content is not None, in which it only matches
non-leaf nodes that also match the content pattern.
The content, if not None, must be a sequence of Patterns that
must match the node's children exactly. If the content is
given, the type must not be None.
If a name is given, the matching node is stored in the results
dict under that key. | __init__ | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def _submatch(self, node, results=None):
"""
Match the pattern's content to the node's children.
This assumes the node type matches and self.content is not None.
Returns True if it matches, False if not.
If results is not None, it must be a dict which will be
updated with the nodes matching named subpatterns.
When returning False, the results dict may still be updated.
"""
if self.wildcards:
for c, r in generate_matches(self.content, node.children):
if c == len(node.children):
if results is not None:
results.update(r)
return True
return False
if len(self.content) != len(node.children):
return False
for subpattern, child in zip(self.content, node.children):
if not subpattern.match(child, results):
return False
return True | Match the pattern's content to the node's children.
This assumes the node type matches and self.content is not None.
Returns True if it matches, False if not.
If results is not None, it must be a dict which will be
updated with the nodes matching named subpatterns.
When returning False, the results dict may still be updated. | _submatch | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def __init__(self, content=None, min=0, max=HUGE, name=None):
"""
Initializer.
Args:
content: optional sequence of subsequences of patterns;
if absent, matches one node;
if present, each subsequence is an alternative [*]
min: optional minimum number of times to match, default 0
max: optional maximum number of times to match, default HUGE
name: optional name assigned to this match
[*] Thus, if content is [[a, b, c], [d, e], [f, g, h]] this is
equivalent to (a b c | d e | f g h); if content is None,
this is equivalent to '.' in regular expression terms.
The min and max parameters work as follows:
min=0, max=maxint: .*
min=1, max=maxint: .+
min=0, max=1: .?
min=1, max=1: .
If content is not None, replace the dot with the parenthesized
list of alternatives, e.g. (a b c | d e | f g h)*
"""
assert 0 <= min <= max <= HUGE, (min, max)
if content is not None:
content = tuple(map(tuple, content)) # Protect against alterations
# Check sanity of alternatives
assert len(content), repr(content) # Can't have zero alternatives
for alt in content:
assert len(alt), repr(alt) # Can have empty alternatives
self.content = content
self.min = min
self.max = max
self.name = name | Initializer.
Args:
content: optional sequence of subsequences of patterns;
if absent, matches one node;
if present, each subsequence is an alternative [*]
min: optional minimum number of times to match, default 0
max: optional maximum number of times to match, default HUGE
name: optional name assigned to this match
[*] Thus, if content is [[a, b, c], [d, e], [f, g, h]] this is
equivalent to (a b c | d e | f g h); if content is None,
this is equivalent to '.' in regular expression terms.
The min and max parameters work as follows:
min=0, max=maxint: .*
min=1, max=maxint: .+
min=0, max=1: .?
min=1, max=1: .
If content is not None, replace the dot with the parenthesized
list of alternatives, e.g. (a b c | d e | f g h)* | __init__ | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
def optimize(self):
"""Optimize certain stacked wildcard patterns."""
subpattern = None
if (self.content is not None and
len(self.content) == 1 and len(self.content[0]) == 1):
subpattern = self.content[0][0]
if self.min == 1 and self.max == 1:
if self.content is None:
return NodePattern(name=self.name)
if subpattern is not None and self.name == subpattern.name:
return subpattern.optimize()
if (self.min <= 1 and isinstance(subpattern, WildcardPattern) and
subpattern.min <= 1 and self.name == subpattern.name):
return WildcardPattern(subpattern.content,
self.min*subpattern.min,
self.max*subpattern.max,
subpattern.name)
return self | Optimize certain stacked wildcard patterns. | optimize | python | sajjadium/ctf-archives | ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | https://github.com/sajjadium/ctf-archives/blob/master/ctfs/TyphoonCon/2022/pwn/beautifier_player/python3.7/lib/python3.7/lib2to3/pytree.py | MIT |
This dataset contains Python functions with their documentation comments extracted from GitHub repositories.
code
: The Python function codedocstring
: Documentation comment for the functionfunc_name
: Function namelanguage
: Programming language (always "Python")repo
: Source repository namepath
: File path within the repositoryurl
: GitHub URL to the source filelicense
: License of the source codeThe dataset contains the following splits:
from datasets import load_dataset
# Load the dataset
dataset = load_dataset("USERNAME/DATASET_NAME")
# Access the training split
train_data = dataset["train"]
# Example: Print the first sample
print(train_data[0])
This dataset was created by scraping Python code from GitHub repositories. Each function includes its documentation comment and license information.
This dataset contains code from various repositories with different licenses. Each sample includes its original license information in the license
field.