function
stringlengths 61
9.1k
| testmethod
stringlengths 42
143
| location_fixed
stringlengths 43
98
| end_buggy
int64 10
12k
| location
stringlengths 43
98
| function_name
stringlengths 4
73
| source_buggy
stringlengths 655
442k
| prompt_complete
stringlengths 433
4.3k
| end_fixed
int64 10
12k
| comment
stringlengths 0
763
| bug_id
stringlengths 1
3
| start_fixed
int64 7
12k
| location_buggy
stringlengths 43
98
| source_dir
stringclasses 5
values | prompt_chat
stringlengths 420
3.86k
| start_buggy
int64 7
12k
| classes_modified
sequence | task_id
stringlengths 64
64
| function_signature
stringlengths 22
150
| prompt_complete_without_signature
stringlengths 404
4.23k
| project
stringclasses 17
values | indent
stringclasses 4
values | source_fixed
stringlengths 655
442k
|
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
public void testIssue794b() {
noInline(
"var x = 1; " +
"try { x = x + someFunction(); } catch (e) {}" +
"x = x + 1;" +
"try { x = x + someFunction(); } catch (e) {}" +
"return x;");
} | com.google.javascript.jscomp.FlowSensitiveInlineVariablesTest::testIssue794b | test/com/google/javascript/jscomp/FlowSensitiveInlineVariablesTest.java | 518 | test/com/google/javascript/jscomp/FlowSensitiveInlineVariablesTest.java | testIssue794b | /*
* Copyright 2009 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import com.google.javascript.rhino.Node;
/**
* Unit tests for {@link FlowSensitiveInlineVariables}.
*
*/
public class FlowSensitiveInlineVariablesTest extends CompilerTestCase {
public static final String EXTERN_FUNCTIONS = "" +
"var print;\n" +
"/** @nosideeffects */ function noSFX() {} \n" +
" function hasSFX() {} \n";
public FlowSensitiveInlineVariablesTest() {
enableNormalize(true);
}
@Override
public int getNumRepetitions() {
// Test repeatedly inline.
return 3;
}
@Override
protected CompilerPass getProcessor(final Compiler compiler) {
//return new FlowSensitiveInlineVariables(compiler);
return new CompilerPass() {
@Override
public void process(Node externs, Node root) {
(new MarkNoSideEffectCalls(compiler)).process(externs, root);
(new FlowSensitiveInlineVariables(compiler)).process(externs, root);
}
};
}
public void testSimpleAssign() {
inline("var x; x = 1; print(x)", "var x; print(1)");
inline("var x; x = 1; x", "var x; 1");
inline("var x; x = 1; var a = x", "var x; var a = 1");
inline("var x; x = 1; x = x + 1", "var x; x = 1 + 1");
}
public void testSimpleVar() {
inline("var x = 1; print(x)", "var x; print(1)");
inline("var x = 1; x", "var x; 1");
inline("var x = 1; var a = x", "var x; var a = 1");
inline("var x = 1; x = x + 1", "var x; x = 1 + 1");
}
public void testSimpleForIn() {
inline("var a,b,x = a in b; x",
"var a,b,x; a in b");
noInline("var a, b; var x = a in b; print(1); x");
noInline("var a,b,x = a in b; delete a[b]; x");
}
public void testExported() {
noInline("var _x = 1; print(_x)");
}
public void testDoNotInlineIncrement() {
noInline("var x = 1; x++;");
noInline("var x = 1; x--;");
}
public void testDoNotInlineAssignmentOp() {
noInline("var x = 1; x += 1;");
noInline("var x = 1; x -= 1;");
}
public void testDoNotInlineIntoLhsOfAssign() {
noInline("var x = 1; x += 3;");
}
public void testMultiUse() {
noInline("var x; x = 1; print(x); print (x);");
}
public void testMultiUseInSameCfgNode() {
noInline("var x; x = 1; print(x) || print (x);");
}
public void testMultiUseInTwoDifferentPath() {
noInline("var x = 1; if (print) { print(x) } else { alert(x) }");
}
public void testAssignmentBeforeDefinition() {
inline("x = 1; var x = 0; print(x)","x = 1; var x; print(0)" );
}
public void testVarInConditionPath() {
noInline("if (foo) { var x = 0 } print(x)");
}
public void testMultiDefinitionsBeforeUse() {
inline("var x = 0; x = 1; print(x)", "var x = 0; print(1)");
}
public void testMultiDefinitionsInSameCfgNode() {
noInline("var x; (x = 1) || (x = 2); print(x)");
noInline("var x; x = (1 || (x = 2)); print(x)");
noInline("var x;(x = 1) && (x = 2); print(x)");
noInline("var x;x = (1 && (x = 2)); print(x)");
noInline("var x; x = 1 , x = 2; print(x)");
}
public void testNotReachingDefinitions() {
noInline("var x; if (foo) { x = 0 } print (x)");
}
public void testNoInlineLoopCarriedDefinition() {
// First print is undefined instead.
noInline("var x; while(true) { print(x); x = 1; }");
// Prints 0 1 1 1 1....
noInline("var x = 0; while(true) { print(x); x = 1; }");
}
public void testDoNotExitLoop() {
noInline("while (z) { var x = 3; } var y = x;");
}
public void testDoNotInlineWithinLoop() {
noInline("var y = noSFX(); do { var z = y.foo(); } while (true);");
}
public void testDefinitionAfterUse() {
inline("var x = 0; print(x); x = 1", "var x; print(0); x = 1");
}
public void testInlineSameVariableInStraightLine() {
inline("var x; x = 1; print(x); x = 2; print(x)",
"var x; print(1); print(2)");
}
public void testInlineInDifferentPaths() {
inline("var x; if (print) {x = 1; print(x)} else {x = 2; print(x)}",
"var x; if (print) {print(1)} else {print(2)}");
}
public void testNoInlineInMergedPath() {
noInline(
"var x,y;x = 1;while(y) { if(y){ print(x) } else { x = 1 } } print(x)");
}
public void testInlineIntoExpressions() {
inline("var x = 1; print(x + 1);", "var x; print(1 + 1)");
}
public void testInlineExpressions1() {
inline("var a, b; var x = a+b; print(x)", "var a, b; var x; print(a+b)");
}
public void testInlineExpressions2() {
// We can't inline because of the redefinition of "a".
noInline("var a, b; var x = a + b; a = 1; print(x)");
}
public void testInlineExpressions3() {
inline("var a,b,x; x=a+b; x=a-b ; print(x)",
"var a,b,x; x=a+b; print(a-b)");
}
public void testInlineExpressions4() {
// Precision is lost due to comma's.
noInline("var a,b,x; x=a+b, x=a-b; print(x)");
}
public void testInlineExpressions5() {
noInline("var a; var x = a = 1; print(x)");
}
public void testInlineExpressions6() {
noInline("var a, x; a = 1 + (x = 1); print(x)");
}
public void testInlineExpression7() {
// Possible side effects in foo() that might conflict with bar();
noInline("var x = foo() + 1; bar(); print(x)");
// This is a possible case but we don't have analysis to prove this yet.
// TODO(user): It is possible to cover this case with the same algorithm
// as the missing return check.
noInline("var x = foo() + 1; print(x)");
}
public void testInlineExpression8() {
// The same variable inlined twice.
inline(
"var a,b;" +
"var x = a + b; print(x); x = a - b; print(x)",
"var a,b;" +
"var x; print(a + b); print(a - b)");
}
public void testInlineExpression9() {
// Check for actual control flow sensitivity.
inline(
"var a,b;" +
"var x; if (g) { x= a + b; print(x) } x = a - b; print(x)",
"var a,b;" +
"var x; if (g) { print(a + b)} print(a - b)");
}
public void testInlineExpression10() {
// The DFA is not fine grain enough for this.
noInline("var x, y; x = ((y = 1), print(y))");
}
public void testInlineExpressions11() {
inline("var x; x = x + 1; print(x)", "var x; print(x + 1)");
noInline("var x; x = x + 1; print(x); print(x)");
}
public void testInlineExpressions12() {
// ++ is an assignment and considered to modify state so it will not be
// inlined.
noInline("var x = 10; x = c++; print(x)");
}
public void testInlineExpressions13() {
inline("var a = 1, b = 2;" +
"var x = a;" +
"var y = b;" +
"var z = x + y;" +
"var i = z;" +
"var j = z + y;" +
"var k = i;",
"var a, b;" +
"var x;" +
"var y = 2;" +
"var z = 1 + y;" +
"var i;" +
"var j = z + y;" +
"var k = z;");
}
public void testNoInlineIfDefinitionMayNotReach() {
noInline("var x; if (x=1) {} x;");
}
public void testNoInlineEscapedToInnerFunction() {
noInline("var x = 1; function foo() { x = 2 }; print(x)");
}
public void testNoInlineLValue() {
noInline("var x; if (x = 1) { print(x) }");
}
public void testSwitchCase() {
inline("var x = 1; switch(x) { }", "var x; switch(1) { }");
}
public void testShadowedVariableInnerFunction() {
inline("var x = 1; print(x) || (function() { var x; x = 1; print(x)})()",
"var x; print(1) || (function() { var x; print(1)})()");
}
public void testCatch() {
noInline("var x = 0; try { } catch (x) { }");
noInline("try { } catch (x) { print(x) }");
}
public void testNoInlineGetProp() {
// We don't know if j alias a.b
noInline("var x = a.b.c; j.c = 1; print(x);");
}
public void testNoInlineGetProp2() {
noInline("var x = 1 * a.b.c; j.c = 1; print(x);");
}
public void testNoInlineGetProp3() {
// Anything inside a function is fine.
inline("var x = function(){1 * a.b.c}; print(x);",
"var x; print(function(){1 * a.b.c});");
}
public void testNoInlineGetEle() {
// Again we don't know if i = j
noInline("var x = a[i]; a[j] = 2; print(x); ");
}
// TODO(user): These should be inlinable.
public void testNoInlineConstructors() {
noInline("var x = new Iterator(); x.next();");
}
// TODO(user): These should be inlinable.
public void testNoInlineArrayLits() {
noInline("var x = []; print(x)");
}
// TODO(user): These should be inlinable.
public void testNoInlineObjectLits() {
noInline("var x = {}; print(x)");
}
// TODO(user): These should be inlinable after the REGEX checks.
public void testNoInlineRegExpLits() {
noInline("var x = /y/; print(x)");
}
public void testInlineConstructorCallsIntoLoop() {
// Don't inline construction into loops.
noInline("var x = new Iterator();" +
"for(i = 0; i < 10; i++) {j = x.next()}");
}
public void testRemoveWithLabels() {
inline("var x = 1; L: x = 2; print(x)", "var x = 1; L:{} print(2)");
inline("var x = 1; L: M: x = 2; print(x)", "var x = 1; L:M:{} print(2)");
inline("var x = 1; L: M: N: x = 2; print(x)",
"var x = 1; L:M:N:{} print(2)");
}
public void testInlineAcrossSideEffect1() {
// This can't be inlined because print() has side-effects and might change
// the definition of noSFX.
//
// noSFX must be both const and pure in order to inline it.
noInline("var y; var x = noSFX(y); print(x)");
//inline("var y; var x = noSFX(y); print(x)", "var y;var x;print(noSFX(y))");
}
public void testInlineAcrossSideEffect2() {
// Think noSFX() as a function that reads y.foo and return it
// and SFX() write some new value of y.foo. If that's the case,
// inlining across hasSFX() is not valid.
// This is a case where hasSFX is right of the source of the inlining.
noInline("var y; var x = noSFX(y), z = hasSFX(y); print(x)");
noInline("var y; var x = noSFX(y), z = new hasSFX(y); print(x)");
noInline("var y; var x = new noSFX(y), z = new hasSFX(y); print(x)");
}
public void testInlineAcrossSideEffect3() {
// This is a case where hasSFX is left of the destination of the inlining.
noInline("var y; var x = noSFX(y); hasSFX(y), print(x)");
noInline("var y; var x = noSFX(y); new hasSFX(y), print(x)");
noInline("var y; var x = new noSFX(y); new hasSFX(y), print(x)");
}
public void testInlineAcrossSideEffect4() {
// This is a case where hasSFX is some control flow path between the
// source and its destination.
noInline("var y; var x = noSFX(y); hasSFX(y); print(x)");
noInline("var y; var x = noSFX(y); new hasSFX(y); print(x)");
noInline("var y; var x = new noSFX(y); new hasSFX(y); print(x)");
}
public void testCanInlineAcrossNoSideEffect() {
// This can't be inlined because print() has side-effects and might change
// the definition of noSFX. We should be able to mark noSFX as const
// in some way.
noInline(
"var y; var x = noSFX(y), z = noSFX(); noSFX(); noSFX(), print(x)");
//inline(
// "var y; var x = noSFX(y), z = noSFX(); noSFX(); noSFX(), print(x)",
// "var y; var x, z = noSFX(); noSFX(); noSFX(), print(noSFX(y))");
}
public void testDependOnOuterScopeVariables() {
noInline("var x; function foo() { var y = x; x = 0; print(y) }");
noInline("var x; function foo() { var y = x; x++; print(y) }");
// Sadly, we don't understand the data flow of outer scoped variables as
// it can be modified by code outside of this scope. We can't inline
// at all if the definition has dependence on such variable.
noInline("var x; function foo() { var y = x; print(y) }");
}
public void testInlineIfNameIsLeftSideOfAssign() {
inline("var x = 1; x = print(x) + 1", "var x; x = print(1) + 1");
inline("var x = 1; L: x = x + 2", "var x; L: x = 1 + 2");
inline("var x = 1; x = (x = x + 1)", "var x; x = (x = 1 + 1)");
noInline("var x = 1; x = (x = (x = 10) + x)");
noInline("var x = 1; x = (f(x) + (x = 10) + x);");
noInline("var x = 1; x=-1,foo(x)");
noInline("var x = 1; x-=1,foo(x)");
}
public void testInlineArguments() {
testSame("function _func(x) { print(x) }");
testSame("function _func(x,y) { if(y) { x = 1 }; print(x) }");
test("function f(x, y) { x = 1; print(x) }",
"function f(x, y) { print(1) }");
test("function f(x, y) { if (y) { x = 1; print(x) }}",
"function f(x, y) { if (y) { print(1) }}");
}
public void testInvalidInlineArguments1() {
testSame("function f(x, y) { x = 1; arguments[0] = 2; print(x) }");
testSame("function f(x, y) { x = 1; var z = arguments;" +
"z[0] = 2; z[1] = 3; print(x)}");
testSame("function g(a){a[0]=2} function f(x){x=1;g(arguments);print(x)}");
}
public void testInvalidInlineArguments2() {
testSame("function f(c) {var f = c; arguments[0] = this;" +
"f.apply(this, arguments); return this;}");
}
public void testForIn() {
noInline("var x; var y = {}; for(x in y){}");
noInline("var x; var y = {}; var z; for(x in z = y){print(z)}");
noInline("var x; var y = {}; var z; for(x in y){print(z)}");
}
public void testNotOkToSkipCheckPathBetweenNodes() {
noInline("var x; for(x = 1; foo(x);) {}");
noInline("var x; for(; x = 1;foo(x)) {}");
}
public void testIssue698() {
// Most of the flow algorithms operate on Vars. We want to make
// sure the algorithm bails out appropriately if it sees
// a var that it doesn't know about.
inline(
"var x = ''; "
+ "unknown.length < 2 && (unknown='0' + unknown);"
+ "x = x + unknown; "
+ "unknown.length < 3 && (unknown='0' + unknown);"
+ "x = x + unknown; "
+ "return x;",
"var x; "
+ "unknown.length < 2 && (unknown='0' + unknown);"
+ "x = '' + unknown; "
+ "unknown.length < 3 && (unknown='0' + unknown);"
+ "x = x + unknown; "
+ "return x;");
}
public void testIssue777() {
test(
"function f(cmd, ta) {" +
" var temp = cmd;" +
" var temp2 = temp >> 2;" +
" cmd = STACKTOP;" +
" for (var src = temp2, dest = cmd >> 2, stop = src + 37;" +
" src < stop;" +
" src++, dest++) {" +
" HEAP32[dest] = HEAP32[src];" +
" }" +
" temp = ta;" +
" temp2 = temp >> 2;" +
" ta = STACKTOP;" +
" STACKTOP += 8;" +
" HEAP32[ta >> 2] = HEAP32[temp2];" +
" HEAP32[ta + 4 >> 2] = HEAP32[temp2 + 1];" +
"}",
"function f(cmd, ta){" +
" var temp;" +
" var temp2 = cmd >> 2;" +
" cmd = STACKTOP;" +
" var src = temp2;" +
" var dest = cmd >> 2;" +
" var stop = src + 37;" +
" for(;src<stop;src++,dest++)HEAP32[dest]=HEAP32[src];" +
" temp2 = ta >> 2;" +
" ta = STACKTOP;" +
" STACKTOP += 8;" +
" HEAP32[ta>>2] = HEAP32[temp2];" +
" HEAP32[ta+4>>2] = HEAP32[temp2+1];" +
"}");
}
public void testTransitiveDependencies1() {
test(
"function f(x) { var a = x; var b = a; x = 3; return b; }",
"function f(x) { var a; var b = x; x = 3; return b; }");
}
public void testTransitiveDependencies2() {
test(
"function f(x) { var a = x; var b = a; var c = b; x = 3; return c; }",
"function f(x) { var a ; var b = x; var c ; x = 3; return b; }");
}
public void testIssue794a() {
noInline(
"var x = 1; " +
"try { x += someFunction(); } catch (e) {}" +
"x += 1;" +
"try { x += someFunction(); } catch (e) {}" +
"return x;");
}
public void testIssue794b() {
noInline(
"var x = 1; " +
"try { x = x + someFunction(); } catch (e) {}" +
"x = x + 1;" +
"try { x = x + someFunction(); } catch (e) {}" +
"return x;");
}
private void noInline(String input) {
inline(input, input);
}
private void inline(String input, String expected) {
test(EXTERN_FUNCTIONS, "function _func() {" + input + "}",
"function _func() {" + expected + "}", null, null);
}
} | // You are a professional Java test case writer, please create a test case named `testIssue794b` for the issue `Closure-794`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-794
//
// ## Issue-Title:
// Try/catch blocks incorporate code not inside original blocks
//
// ## Issue-Description:
// **What steps will reproduce the problem?**
//
// Starting with this code:
//
// -----
// function a() {
// var x = '1';
// try {
// x += somefunction();
// } catch(e) {
// }
// x += "2";
// try {
// x += somefunction();
// } catch(e) {
// }
// document.write(x);
// }
//
// a();
// a();
// -----
//
// It gets compiled to:
//
// -----
// function b() {
// var a;
// try {
// a = "1" + somefunction()
// }catch(c) {
// }
// try {
// a = a + "2" + somefunction()
// }catch(d) {
// }
// document.write(a)
// }
// b();
// b();
// -----
//
// **What is the expected output? What do you see instead?**
//
// The problem is that it's including the constant "1" and "2" inside the try block when the shouldn't be. When executed uncompiled, the script prints "1212". When compiled, the script prints "undefinedundefined".
//
// This behavior doesn't happen if the entire function gets inlined, or if the code between the two try blocks is sufficiently complex.
//
//
// **What version of the product are you using? On what operating system?**
//
// Closure Compiler (http://code.google.com/closure/compiler)
// Version: 20120430 (revision 1918)
// Built on: 2012/04/30 18:02
// java version "1.6.0\_33"
// Java(TM) SE Runtime Environment (build 1.6.0\_33-b03-424-11M3720)
// Java HotSpot(TM) 64-Bit Server VM (build 20.8-b03-424, mixed mode)
//
//
public void testIssue794b() {
| 518 | 12 | 511 | test/com/google/javascript/jscomp/FlowSensitiveInlineVariablesTest.java | test | ```markdown
## Issue-ID: Closure-794
## Issue-Title:
Try/catch blocks incorporate code not inside original blocks
## Issue-Description:
**What steps will reproduce the problem?**
Starting with this code:
-----
function a() {
var x = '1';
try {
x += somefunction();
} catch(e) {
}
x += "2";
try {
x += somefunction();
} catch(e) {
}
document.write(x);
}
a();
a();
-----
It gets compiled to:
-----
function b() {
var a;
try {
a = "1" + somefunction()
}catch(c) {
}
try {
a = a + "2" + somefunction()
}catch(d) {
}
document.write(a)
}
b();
b();
-----
**What is the expected output? What do you see instead?**
The problem is that it's including the constant "1" and "2" inside the try block when the shouldn't be. When executed uncompiled, the script prints "1212". When compiled, the script prints "undefinedundefined".
This behavior doesn't happen if the entire function gets inlined, or if the code between the two try blocks is sufficiently complex.
**What version of the product are you using? On what operating system?**
Closure Compiler (http://code.google.com/closure/compiler)
Version: 20120430 (revision 1918)
Built on: 2012/04/30 18:02
java version "1.6.0\_33"
Java(TM) SE Runtime Environment (build 1.6.0\_33-b03-424-11M3720)
Java HotSpot(TM) 64-Bit Server VM (build 20.8-b03-424, mixed mode)
```
You are a professional Java test case writer, please create a test case named `testIssue794b` for the issue `Closure-794`, utilizing the provided issue report information and the following function signature.
```java
public void testIssue794b() {
```
| 511 | [
"com.google.javascript.jscomp.MaybeReachingVariableUse"
] | 6c69251b3fd7b4a04003663fa13b311a08fa7d782c5577615e78146bd6ad0236 | public void testIssue794b() | // You are a professional Java test case writer, please create a test case named `testIssue794b` for the issue `Closure-794`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-794
//
// ## Issue-Title:
// Try/catch blocks incorporate code not inside original blocks
//
// ## Issue-Description:
// **What steps will reproduce the problem?**
//
// Starting with this code:
//
// -----
// function a() {
// var x = '1';
// try {
// x += somefunction();
// } catch(e) {
// }
// x += "2";
// try {
// x += somefunction();
// } catch(e) {
// }
// document.write(x);
// }
//
// a();
// a();
// -----
//
// It gets compiled to:
//
// -----
// function b() {
// var a;
// try {
// a = "1" + somefunction()
// }catch(c) {
// }
// try {
// a = a + "2" + somefunction()
// }catch(d) {
// }
// document.write(a)
// }
// b();
// b();
// -----
//
// **What is the expected output? What do you see instead?**
//
// The problem is that it's including the constant "1" and "2" inside the try block when the shouldn't be. When executed uncompiled, the script prints "1212". When compiled, the script prints "undefinedundefined".
//
// This behavior doesn't happen if the entire function gets inlined, or if the code between the two try blocks is sufficiently complex.
//
//
// **What version of the product are you using? On what operating system?**
//
// Closure Compiler (http://code.google.com/closure/compiler)
// Version: 20120430 (revision 1918)
// Built on: 2012/04/30 18:02
// java version "1.6.0\_33"
// Java(TM) SE Runtime Environment (build 1.6.0\_33-b03-424-11M3720)
// Java HotSpot(TM) 64-Bit Server VM (build 20.8-b03-424, mixed mode)
//
//
| Closure | /*
* Copyright 2009 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import com.google.javascript.rhino.Node;
/**
* Unit tests for {@link FlowSensitiveInlineVariables}.
*
*/
public class FlowSensitiveInlineVariablesTest extends CompilerTestCase {
public static final String EXTERN_FUNCTIONS = "" +
"var print;\n" +
"/** @nosideeffects */ function noSFX() {} \n" +
" function hasSFX() {} \n";
public FlowSensitiveInlineVariablesTest() {
enableNormalize(true);
}
@Override
public int getNumRepetitions() {
// Test repeatedly inline.
return 3;
}
@Override
protected CompilerPass getProcessor(final Compiler compiler) {
//return new FlowSensitiveInlineVariables(compiler);
return new CompilerPass() {
@Override
public void process(Node externs, Node root) {
(new MarkNoSideEffectCalls(compiler)).process(externs, root);
(new FlowSensitiveInlineVariables(compiler)).process(externs, root);
}
};
}
public void testSimpleAssign() {
inline("var x; x = 1; print(x)", "var x; print(1)");
inline("var x; x = 1; x", "var x; 1");
inline("var x; x = 1; var a = x", "var x; var a = 1");
inline("var x; x = 1; x = x + 1", "var x; x = 1 + 1");
}
public void testSimpleVar() {
inline("var x = 1; print(x)", "var x; print(1)");
inline("var x = 1; x", "var x; 1");
inline("var x = 1; var a = x", "var x; var a = 1");
inline("var x = 1; x = x + 1", "var x; x = 1 + 1");
}
public void testSimpleForIn() {
inline("var a,b,x = a in b; x",
"var a,b,x; a in b");
noInline("var a, b; var x = a in b; print(1); x");
noInline("var a,b,x = a in b; delete a[b]; x");
}
public void testExported() {
noInline("var _x = 1; print(_x)");
}
public void testDoNotInlineIncrement() {
noInline("var x = 1; x++;");
noInline("var x = 1; x--;");
}
public void testDoNotInlineAssignmentOp() {
noInline("var x = 1; x += 1;");
noInline("var x = 1; x -= 1;");
}
public void testDoNotInlineIntoLhsOfAssign() {
noInline("var x = 1; x += 3;");
}
public void testMultiUse() {
noInline("var x; x = 1; print(x); print (x);");
}
public void testMultiUseInSameCfgNode() {
noInline("var x; x = 1; print(x) || print (x);");
}
public void testMultiUseInTwoDifferentPath() {
noInline("var x = 1; if (print) { print(x) } else { alert(x) }");
}
public void testAssignmentBeforeDefinition() {
inline("x = 1; var x = 0; print(x)","x = 1; var x; print(0)" );
}
public void testVarInConditionPath() {
noInline("if (foo) { var x = 0 } print(x)");
}
public void testMultiDefinitionsBeforeUse() {
inline("var x = 0; x = 1; print(x)", "var x = 0; print(1)");
}
public void testMultiDefinitionsInSameCfgNode() {
noInline("var x; (x = 1) || (x = 2); print(x)");
noInline("var x; x = (1 || (x = 2)); print(x)");
noInline("var x;(x = 1) && (x = 2); print(x)");
noInline("var x;x = (1 && (x = 2)); print(x)");
noInline("var x; x = 1 , x = 2; print(x)");
}
public void testNotReachingDefinitions() {
noInline("var x; if (foo) { x = 0 } print (x)");
}
public void testNoInlineLoopCarriedDefinition() {
// First print is undefined instead.
noInline("var x; while(true) { print(x); x = 1; }");
// Prints 0 1 1 1 1....
noInline("var x = 0; while(true) { print(x); x = 1; }");
}
public void testDoNotExitLoop() {
noInline("while (z) { var x = 3; } var y = x;");
}
public void testDoNotInlineWithinLoop() {
noInline("var y = noSFX(); do { var z = y.foo(); } while (true);");
}
public void testDefinitionAfterUse() {
inline("var x = 0; print(x); x = 1", "var x; print(0); x = 1");
}
public void testInlineSameVariableInStraightLine() {
inline("var x; x = 1; print(x); x = 2; print(x)",
"var x; print(1); print(2)");
}
public void testInlineInDifferentPaths() {
inline("var x; if (print) {x = 1; print(x)} else {x = 2; print(x)}",
"var x; if (print) {print(1)} else {print(2)}");
}
public void testNoInlineInMergedPath() {
noInline(
"var x,y;x = 1;while(y) { if(y){ print(x) } else { x = 1 } } print(x)");
}
public void testInlineIntoExpressions() {
inline("var x = 1; print(x + 1);", "var x; print(1 + 1)");
}
public void testInlineExpressions1() {
inline("var a, b; var x = a+b; print(x)", "var a, b; var x; print(a+b)");
}
public void testInlineExpressions2() {
// We can't inline because of the redefinition of "a".
noInline("var a, b; var x = a + b; a = 1; print(x)");
}
public void testInlineExpressions3() {
inline("var a,b,x; x=a+b; x=a-b ; print(x)",
"var a,b,x; x=a+b; print(a-b)");
}
public void testInlineExpressions4() {
// Precision is lost due to comma's.
noInline("var a,b,x; x=a+b, x=a-b; print(x)");
}
public void testInlineExpressions5() {
noInline("var a; var x = a = 1; print(x)");
}
public void testInlineExpressions6() {
noInline("var a, x; a = 1 + (x = 1); print(x)");
}
public void testInlineExpression7() {
// Possible side effects in foo() that might conflict with bar();
noInline("var x = foo() + 1; bar(); print(x)");
// This is a possible case but we don't have analysis to prove this yet.
// TODO(user): It is possible to cover this case with the same algorithm
// as the missing return check.
noInline("var x = foo() + 1; print(x)");
}
public void testInlineExpression8() {
// The same variable inlined twice.
inline(
"var a,b;" +
"var x = a + b; print(x); x = a - b; print(x)",
"var a,b;" +
"var x; print(a + b); print(a - b)");
}
public void testInlineExpression9() {
// Check for actual control flow sensitivity.
inline(
"var a,b;" +
"var x; if (g) { x= a + b; print(x) } x = a - b; print(x)",
"var a,b;" +
"var x; if (g) { print(a + b)} print(a - b)");
}
public void testInlineExpression10() {
// The DFA is not fine grain enough for this.
noInline("var x, y; x = ((y = 1), print(y))");
}
public void testInlineExpressions11() {
inline("var x; x = x + 1; print(x)", "var x; print(x + 1)");
noInline("var x; x = x + 1; print(x); print(x)");
}
public void testInlineExpressions12() {
// ++ is an assignment and considered to modify state so it will not be
// inlined.
noInline("var x = 10; x = c++; print(x)");
}
public void testInlineExpressions13() {
inline("var a = 1, b = 2;" +
"var x = a;" +
"var y = b;" +
"var z = x + y;" +
"var i = z;" +
"var j = z + y;" +
"var k = i;",
"var a, b;" +
"var x;" +
"var y = 2;" +
"var z = 1 + y;" +
"var i;" +
"var j = z + y;" +
"var k = z;");
}
public void testNoInlineIfDefinitionMayNotReach() {
noInline("var x; if (x=1) {} x;");
}
public void testNoInlineEscapedToInnerFunction() {
noInline("var x = 1; function foo() { x = 2 }; print(x)");
}
public void testNoInlineLValue() {
noInline("var x; if (x = 1) { print(x) }");
}
public void testSwitchCase() {
inline("var x = 1; switch(x) { }", "var x; switch(1) { }");
}
public void testShadowedVariableInnerFunction() {
inline("var x = 1; print(x) || (function() { var x; x = 1; print(x)})()",
"var x; print(1) || (function() { var x; print(1)})()");
}
public void testCatch() {
noInline("var x = 0; try { } catch (x) { }");
noInline("try { } catch (x) { print(x) }");
}
public void testNoInlineGetProp() {
// We don't know if j alias a.b
noInline("var x = a.b.c; j.c = 1; print(x);");
}
public void testNoInlineGetProp2() {
noInline("var x = 1 * a.b.c; j.c = 1; print(x);");
}
public void testNoInlineGetProp3() {
// Anything inside a function is fine.
inline("var x = function(){1 * a.b.c}; print(x);",
"var x; print(function(){1 * a.b.c});");
}
public void testNoInlineGetEle() {
// Again we don't know if i = j
noInline("var x = a[i]; a[j] = 2; print(x); ");
}
// TODO(user): These should be inlinable.
public void testNoInlineConstructors() {
noInline("var x = new Iterator(); x.next();");
}
// TODO(user): These should be inlinable.
public void testNoInlineArrayLits() {
noInline("var x = []; print(x)");
}
// TODO(user): These should be inlinable.
public void testNoInlineObjectLits() {
noInline("var x = {}; print(x)");
}
// TODO(user): These should be inlinable after the REGEX checks.
public void testNoInlineRegExpLits() {
noInline("var x = /y/; print(x)");
}
public void testInlineConstructorCallsIntoLoop() {
// Don't inline construction into loops.
noInline("var x = new Iterator();" +
"for(i = 0; i < 10; i++) {j = x.next()}");
}
public void testRemoveWithLabels() {
inline("var x = 1; L: x = 2; print(x)", "var x = 1; L:{} print(2)");
inline("var x = 1; L: M: x = 2; print(x)", "var x = 1; L:M:{} print(2)");
inline("var x = 1; L: M: N: x = 2; print(x)",
"var x = 1; L:M:N:{} print(2)");
}
public void testInlineAcrossSideEffect1() {
// This can't be inlined because print() has side-effects and might change
// the definition of noSFX.
//
// noSFX must be both const and pure in order to inline it.
noInline("var y; var x = noSFX(y); print(x)");
//inline("var y; var x = noSFX(y); print(x)", "var y;var x;print(noSFX(y))");
}
public void testInlineAcrossSideEffect2() {
// Think noSFX() as a function that reads y.foo and return it
// and SFX() write some new value of y.foo. If that's the case,
// inlining across hasSFX() is not valid.
// This is a case where hasSFX is right of the source of the inlining.
noInline("var y; var x = noSFX(y), z = hasSFX(y); print(x)");
noInline("var y; var x = noSFX(y), z = new hasSFX(y); print(x)");
noInline("var y; var x = new noSFX(y), z = new hasSFX(y); print(x)");
}
public void testInlineAcrossSideEffect3() {
// This is a case where hasSFX is left of the destination of the inlining.
noInline("var y; var x = noSFX(y); hasSFX(y), print(x)");
noInline("var y; var x = noSFX(y); new hasSFX(y), print(x)");
noInline("var y; var x = new noSFX(y); new hasSFX(y), print(x)");
}
public void testInlineAcrossSideEffect4() {
// This is a case where hasSFX is some control flow path between the
// source and its destination.
noInline("var y; var x = noSFX(y); hasSFX(y); print(x)");
noInline("var y; var x = noSFX(y); new hasSFX(y); print(x)");
noInline("var y; var x = new noSFX(y); new hasSFX(y); print(x)");
}
public void testCanInlineAcrossNoSideEffect() {
// This can't be inlined because print() has side-effects and might change
// the definition of noSFX. We should be able to mark noSFX as const
// in some way.
noInline(
"var y; var x = noSFX(y), z = noSFX(); noSFX(); noSFX(), print(x)");
//inline(
// "var y; var x = noSFX(y), z = noSFX(); noSFX(); noSFX(), print(x)",
// "var y; var x, z = noSFX(); noSFX(); noSFX(), print(noSFX(y))");
}
public void testDependOnOuterScopeVariables() {
noInline("var x; function foo() { var y = x; x = 0; print(y) }");
noInline("var x; function foo() { var y = x; x++; print(y) }");
// Sadly, we don't understand the data flow of outer scoped variables as
// it can be modified by code outside of this scope. We can't inline
// at all if the definition has dependence on such variable.
noInline("var x; function foo() { var y = x; print(y) }");
}
public void testInlineIfNameIsLeftSideOfAssign() {
inline("var x = 1; x = print(x) + 1", "var x; x = print(1) + 1");
inline("var x = 1; L: x = x + 2", "var x; L: x = 1 + 2");
inline("var x = 1; x = (x = x + 1)", "var x; x = (x = 1 + 1)");
noInline("var x = 1; x = (x = (x = 10) + x)");
noInline("var x = 1; x = (f(x) + (x = 10) + x);");
noInline("var x = 1; x=-1,foo(x)");
noInline("var x = 1; x-=1,foo(x)");
}
public void testInlineArguments() {
testSame("function _func(x) { print(x) }");
testSame("function _func(x,y) { if(y) { x = 1 }; print(x) }");
test("function f(x, y) { x = 1; print(x) }",
"function f(x, y) { print(1) }");
test("function f(x, y) { if (y) { x = 1; print(x) }}",
"function f(x, y) { if (y) { print(1) }}");
}
public void testInvalidInlineArguments1() {
testSame("function f(x, y) { x = 1; arguments[0] = 2; print(x) }");
testSame("function f(x, y) { x = 1; var z = arguments;" +
"z[0] = 2; z[1] = 3; print(x)}");
testSame("function g(a){a[0]=2} function f(x){x=1;g(arguments);print(x)}");
}
public void testInvalidInlineArguments2() {
testSame("function f(c) {var f = c; arguments[0] = this;" +
"f.apply(this, arguments); return this;}");
}
public void testForIn() {
noInline("var x; var y = {}; for(x in y){}");
noInline("var x; var y = {}; var z; for(x in z = y){print(z)}");
noInline("var x; var y = {}; var z; for(x in y){print(z)}");
}
public void testNotOkToSkipCheckPathBetweenNodes() {
noInline("var x; for(x = 1; foo(x);) {}");
noInline("var x; for(; x = 1;foo(x)) {}");
}
public void testIssue698() {
// Most of the flow algorithms operate on Vars. We want to make
// sure the algorithm bails out appropriately if it sees
// a var that it doesn't know about.
inline(
"var x = ''; "
+ "unknown.length < 2 && (unknown='0' + unknown);"
+ "x = x + unknown; "
+ "unknown.length < 3 && (unknown='0' + unknown);"
+ "x = x + unknown; "
+ "return x;",
"var x; "
+ "unknown.length < 2 && (unknown='0' + unknown);"
+ "x = '' + unknown; "
+ "unknown.length < 3 && (unknown='0' + unknown);"
+ "x = x + unknown; "
+ "return x;");
}
public void testIssue777() {
test(
"function f(cmd, ta) {" +
" var temp = cmd;" +
" var temp2 = temp >> 2;" +
" cmd = STACKTOP;" +
" for (var src = temp2, dest = cmd >> 2, stop = src + 37;" +
" src < stop;" +
" src++, dest++) {" +
" HEAP32[dest] = HEAP32[src];" +
" }" +
" temp = ta;" +
" temp2 = temp >> 2;" +
" ta = STACKTOP;" +
" STACKTOP += 8;" +
" HEAP32[ta >> 2] = HEAP32[temp2];" +
" HEAP32[ta + 4 >> 2] = HEAP32[temp2 + 1];" +
"}",
"function f(cmd, ta){" +
" var temp;" +
" var temp2 = cmd >> 2;" +
" cmd = STACKTOP;" +
" var src = temp2;" +
" var dest = cmd >> 2;" +
" var stop = src + 37;" +
" for(;src<stop;src++,dest++)HEAP32[dest]=HEAP32[src];" +
" temp2 = ta >> 2;" +
" ta = STACKTOP;" +
" STACKTOP += 8;" +
" HEAP32[ta>>2] = HEAP32[temp2];" +
" HEAP32[ta+4>>2] = HEAP32[temp2+1];" +
"}");
}
public void testTransitiveDependencies1() {
test(
"function f(x) { var a = x; var b = a; x = 3; return b; }",
"function f(x) { var a; var b = x; x = 3; return b; }");
}
public void testTransitiveDependencies2() {
test(
"function f(x) { var a = x; var b = a; var c = b; x = 3; return c; }",
"function f(x) { var a ; var b = x; var c ; x = 3; return b; }");
}
public void testIssue794a() {
noInline(
"var x = 1; " +
"try { x += someFunction(); } catch (e) {}" +
"x += 1;" +
"try { x += someFunction(); } catch (e) {}" +
"return x;");
}
public void testIssue794b() {
noInline(
"var x = 1; " +
"try { x = x + someFunction(); } catch (e) {}" +
"x = x + 1;" +
"try { x = x + someFunction(); } catch (e) {}" +
"return x;");
}
private void noInline(String input) {
inline(input, input);
}
private void inline(String input, String expected) {
test(EXTERN_FUNCTIONS, "function _func() {" + input + "}",
"function _func() {" + expected + "}", null, null);
}
} |
||
public void testWith3() {
Partial test = createHourMinPartial();
try {
test.with(DateTimeFieldType.clockhourOfDay(), 6);
fail();
} catch (IllegalArgumentException ex) {}
check(test, 10, 20);
} | org.joda.time.TestPartial_Basics::testWith3 | src/test/java/org/joda/time/TestPartial_Basics.java | 366 | src/test/java/org/joda/time/TestPartial_Basics.java | testWith3 | /*
* Copyright 2001-2013 Stephen Colebourne
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.joda.time;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.util.Arrays;
import java.util.Locale;
import junit.framework.TestCase;
import junit.framework.TestSuite;
import org.joda.time.chrono.BuddhistChronology;
import org.joda.time.chrono.CopticChronology;
import org.joda.time.chrono.ISOChronology;
import org.joda.time.format.DateTimeFormat;
import org.joda.time.format.DateTimeFormatter;
/**
* This class is a Junit unit test for Partial.
*
* @author Stephen Colebourne
*/
public class TestPartial_Basics extends TestCase {
private static final DateTimeZone PARIS = DateTimeZone.forID("Europe/Paris");
private static final DateTimeZone LONDON = DateTimeZone.forID("Europe/London");
private static final DateTimeZone TOKYO = DateTimeZone.forID("Asia/Tokyo");
private static final Chronology COPTIC_PARIS = CopticChronology.getInstance(PARIS);
private static final Chronology COPTIC_TOKYO = CopticChronology.getInstance(TOKYO);
private static final Chronology COPTIC_UTC = CopticChronology.getInstanceUTC();
private static final Chronology ISO_UTC = ISOChronology.getInstanceUTC();
private static final Chronology BUDDHIST_LONDON = BuddhistChronology.getInstance(LONDON);
private static final Chronology BUDDHIST_TOKYO = BuddhistChronology.getInstance(TOKYO);
private static final Chronology BUDDHIST_UTC = BuddhistChronology.getInstanceUTC();
private long TEST_TIME_NOW =
10L * DateTimeConstants.MILLIS_PER_HOUR
+ 20L * DateTimeConstants.MILLIS_PER_MINUTE
+ 30L * DateTimeConstants.MILLIS_PER_SECOND
+ 40L;
private long TEST_TIME2 =
1L * DateTimeConstants.MILLIS_PER_DAY
+ 5L * DateTimeConstants.MILLIS_PER_HOUR
+ 6L * DateTimeConstants.MILLIS_PER_MINUTE
+ 7L * DateTimeConstants.MILLIS_PER_SECOND
+ 8L;
private DateTimeZone zone = null;
public static void main(String[] args) {
junit.textui.TestRunner.run(suite());
}
public static TestSuite suite() {
return new TestSuite(TestPartial_Basics.class);
}
public TestPartial_Basics(String name) {
super(name);
}
protected void setUp() throws Exception {
DateTimeUtils.setCurrentMillisFixed(TEST_TIME_NOW);
zone = DateTimeZone.getDefault();
DateTimeZone.setDefault(LONDON);
}
protected void tearDown() throws Exception {
DateTimeUtils.setCurrentMillisSystem();
DateTimeZone.setDefault(zone);
zone = null;
}
//-----------------------------------------------------------------------
public void testGet() {
Partial test = createHourMinPartial();
assertEquals(10, test.get(DateTimeFieldType.hourOfDay()));
assertEquals(20, test.get(DateTimeFieldType.minuteOfHour()));
try {
test.get(null);
fail();
} catch (IllegalArgumentException ex) {}
try {
test.get(DateTimeFieldType.secondOfMinute());
fail();
} catch (IllegalArgumentException ex) {}
}
public void testSize() {
Partial test = createHourMinPartial();
assertEquals(2, test.size());
}
public void testGetFieldType() {
Partial test = createHourMinPartial();
assertSame(DateTimeFieldType.hourOfDay(), test.getFieldType(0));
assertSame(DateTimeFieldType.minuteOfHour(), test.getFieldType(1));
try {
test.getFieldType(-1);
} catch (IndexOutOfBoundsException ex) {}
try {
test.getFieldType(2);
} catch (IndexOutOfBoundsException ex) {}
}
public void testGetFieldTypes() {
Partial test = createHourMinPartial();
DateTimeFieldType[] fields = test.getFieldTypes();
assertEquals(2, fields.length);
assertSame(DateTimeFieldType.hourOfDay(), fields[0]);
assertSame(DateTimeFieldType.minuteOfHour(), fields[1]);
assertNotSame(test.getFieldTypes(), test.getFieldTypes());
}
public void testGetField() {
Partial test = createHourMinPartial(COPTIC_PARIS);
assertSame(CopticChronology.getInstanceUTC().hourOfDay(), test.getField(0));
assertSame(CopticChronology.getInstanceUTC().minuteOfHour(), test.getField(1));
try {
test.getField(-1);
} catch (IndexOutOfBoundsException ex) {}
try {
test.getField(5);
} catch (IndexOutOfBoundsException ex) {}
}
public void testGetFields() {
Partial test = createHourMinPartial(COPTIC_PARIS);
DateTimeField[] fields = test.getFields();
assertEquals(2, fields.length);
assertSame(CopticChronology.getInstanceUTC().hourOfDay(), fields[0]);
assertSame(CopticChronology.getInstanceUTC().minuteOfHour(), fields[1]);
assertNotSame(test.getFields(), test.getFields());
}
public void testGetValue() {
Partial test = createHourMinPartial(COPTIC_PARIS);
assertEquals(10, test.getValue(0));
assertEquals(20, test.getValue(1));
try {
test.getValue(-1);
} catch (IndexOutOfBoundsException ex) {}
try {
test.getValue(2);
} catch (IndexOutOfBoundsException ex) {}
}
public void testGetValues() {
Partial test = createHourMinPartial(COPTIC_PARIS);
int[] values = test.getValues();
assertEquals(2, values.length);
assertEquals(10, values[0]);
assertEquals(20, values[1]);
assertNotSame(test.getValues(), test.getValues());
}
public void testIsSupported() {
Partial test = createHourMinPartial(COPTIC_PARIS);
assertEquals(true, test.isSupported(DateTimeFieldType.hourOfDay()));
assertEquals(true, test.isSupported(DateTimeFieldType.minuteOfHour()));
assertEquals(false, test.isSupported(DateTimeFieldType.secondOfMinute()));
assertEquals(false, test.isSupported(DateTimeFieldType.millisOfSecond()));
assertEquals(false, test.isSupported(DateTimeFieldType.dayOfMonth()));
}
@SuppressWarnings("deprecation")
public void testEqualsHashCode() {
Partial test1 = createHourMinPartial(COPTIC_PARIS);
Partial test2 = createHourMinPartial(COPTIC_PARIS);
assertEquals(true, test1.equals(test2));
assertEquals(true, test2.equals(test1));
assertEquals(true, test1.equals(test1));
assertEquals(true, test2.equals(test2));
assertEquals(true, test1.hashCode() == test2.hashCode());
assertEquals(true, test1.hashCode() == test1.hashCode());
assertEquals(true, test2.hashCode() == test2.hashCode());
Partial test3 = createHourMinPartial2(COPTIC_PARIS);
assertEquals(false, test1.equals(test3));
assertEquals(false, test2.equals(test3));
assertEquals(false, test3.equals(test1));
assertEquals(false, test3.equals(test2));
assertEquals(false, test1.hashCode() == test3.hashCode());
assertEquals(false, test2.hashCode() == test3.hashCode());
assertEquals(false, test1.equals("Hello"));
assertEquals(false, test1.equals(MockPartial.EMPTY_INSTANCE));
assertEquals(new TimeOfDay(10, 20, 30, 40), createTODPartial(ISO_UTC));
}
//-----------------------------------------------------------------------
@SuppressWarnings("deprecation")
public void testCompareTo() {
Partial test1 = createHourMinPartial();
Partial test1a = createHourMinPartial();
assertEquals(0, test1.compareTo(test1a));
assertEquals(0, test1a.compareTo(test1));
assertEquals(0, test1.compareTo(test1));
assertEquals(0, test1a.compareTo(test1a));
Partial test2 = createHourMinPartial2(ISO_UTC);
assertEquals(-1, test1.compareTo(test2));
assertEquals(+1, test2.compareTo(test1));
Partial test3 = createHourMinPartial2(COPTIC_UTC);
assertEquals(-1, test1.compareTo(test3));
assertEquals(+1, test3.compareTo(test1));
assertEquals(0, test3.compareTo(test2));
assertEquals(0, new TimeOfDay(10, 20, 30, 40).compareTo(createTODPartial(ISO_UTC)));
try {
test1.compareTo(null);
fail();
} catch (NullPointerException ex) {}
// try {
// test1.compareTo(new Date());
// fail();
// } catch (ClassCastException ex) {}
try {
test1.compareTo(new YearMonthDay());
fail();
} catch (ClassCastException ex) {}
try {
createTODPartial(ISO_UTC).without(DateTimeFieldType.hourOfDay()).compareTo(new YearMonthDay());
fail();
} catch (ClassCastException ex) {}
}
//-----------------------------------------------------------------------
public void testIsEqual_TOD() {
Partial test1 = createHourMinPartial();
Partial test1a = createHourMinPartial();
assertEquals(true, test1.isEqual(test1a));
assertEquals(true, test1a.isEqual(test1));
assertEquals(true, test1.isEqual(test1));
assertEquals(true, test1a.isEqual(test1a));
Partial test2 = createHourMinPartial2(ISO_UTC);
assertEquals(false, test1.isEqual(test2));
assertEquals(false, test2.isEqual(test1));
Partial test3 = createHourMinPartial2(COPTIC_UTC);
assertEquals(false, test1.isEqual(test3));
assertEquals(false, test3.isEqual(test1));
assertEquals(true, test3.isEqual(test2));
try {
createHourMinPartial().isEqual(null);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testIsBefore_TOD() {
Partial test1 = createHourMinPartial();
Partial test1a = createHourMinPartial();
assertEquals(false, test1.isBefore(test1a));
assertEquals(false, test1a.isBefore(test1));
assertEquals(false, test1.isBefore(test1));
assertEquals(false, test1a.isBefore(test1a));
Partial test2 = createHourMinPartial2(ISO_UTC);
assertEquals(true, test1.isBefore(test2));
assertEquals(false, test2.isBefore(test1));
Partial test3 = createHourMinPartial2(COPTIC_UTC);
assertEquals(true, test1.isBefore(test3));
assertEquals(false, test3.isBefore(test1));
assertEquals(false, test3.isBefore(test2));
try {
createHourMinPartial().isBefore(null);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testIsAfter_TOD() {
Partial test1 = createHourMinPartial();
Partial test1a = createHourMinPartial();
assertEquals(false, test1.isAfter(test1a));
assertEquals(false, test1a.isAfter(test1));
assertEquals(false, test1.isAfter(test1));
assertEquals(false, test1a.isAfter(test1a));
Partial test2 = createHourMinPartial2(ISO_UTC);
assertEquals(false, test1.isAfter(test2));
assertEquals(true, test2.isAfter(test1));
Partial test3 = createHourMinPartial2(COPTIC_UTC);
assertEquals(false, test1.isAfter(test3));
assertEquals(true, test3.isAfter(test1));
assertEquals(false, test3.isAfter(test2));
try {
createHourMinPartial().isAfter(null);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testWithChronologyRetainFields_Chrono() {
Partial base = createHourMinPartial(COPTIC_PARIS);
Partial test = base.withChronologyRetainFields(BUDDHIST_TOKYO);
check(base, 10, 20);
assertEquals(COPTIC_UTC, base.getChronology());
check(test, 10, 20);
assertEquals(BUDDHIST_UTC, test.getChronology());
}
public void testWithChronologyRetainFields_sameChrono() {
Partial base = createHourMinPartial(COPTIC_PARIS);
Partial test = base.withChronologyRetainFields(COPTIC_TOKYO);
assertSame(base, test);
}
public void testWithChronologyRetainFields_nullChrono() {
Partial base = createHourMinPartial(COPTIC_PARIS);
Partial test = base.withChronologyRetainFields(null);
check(base, 10, 20);
assertEquals(COPTIC_UTC, base.getChronology());
check(test, 10, 20);
assertEquals(ISO_UTC, test.getChronology());
}
//-----------------------------------------------------------------------
public void testWith1() {
Partial test = createHourMinPartial();
Partial result = test.with(DateTimeFieldType.hourOfDay(), 15);
check(test, 10, 20);
check(result, 15, 20);
}
public void testWith2() {
Partial test = createHourMinPartial();
try {
test.with(null, 6);
fail();
} catch (IllegalArgumentException ex) {}
check(test, 10, 20);
}
public void testWith3() {
Partial test = createHourMinPartial();
try {
test.with(DateTimeFieldType.clockhourOfDay(), 6);
fail();
} catch (IllegalArgumentException ex) {}
check(test, 10, 20);
}
public void testWith3a() {
Partial test = createHourMinPartial();
Partial result = test.with(DateTimeFieldType.secondOfMinute(), 15);
check(test, 10, 20);
assertEquals(3, result.size());
assertEquals(true, result.isSupported(DateTimeFieldType.hourOfDay()));
assertEquals(true, result.isSupported(DateTimeFieldType.minuteOfHour()));
assertEquals(true, result.isSupported(DateTimeFieldType.secondOfMinute()));
assertEquals(DateTimeFieldType.hourOfDay(), result.getFieldType(0));
assertEquals(DateTimeFieldType.minuteOfHour(), result.getFieldType(1));
assertEquals(DateTimeFieldType.secondOfMinute(), result.getFieldType(2));
assertEquals(10, result.get(DateTimeFieldType.hourOfDay()));
assertEquals(20, result.get(DateTimeFieldType.minuteOfHour()));
assertEquals(15, result.get(DateTimeFieldType.secondOfMinute()));
}
public void testWith3b() {
Partial test = createHourMinPartial();
Partial result = test.with(DateTimeFieldType.minuteOfDay(), 15);
check(test, 10, 20);
assertEquals(3, result.size());
assertEquals(true, result.isSupported(DateTimeFieldType.hourOfDay()));
assertEquals(true, result.isSupported(DateTimeFieldType.minuteOfDay()));
assertEquals(true, result.isSupported(DateTimeFieldType.minuteOfHour()));
assertEquals(DateTimeFieldType.hourOfDay(), result.getFieldType(0));
assertEquals(DateTimeFieldType.minuteOfDay(), result.getFieldType(1));
assertEquals(DateTimeFieldType.minuteOfHour(), result.getFieldType(2));
assertEquals(10, result.get(DateTimeFieldType.hourOfDay()));
assertEquals(20, result.get(DateTimeFieldType.minuteOfHour()));
assertEquals(15, result.get(DateTimeFieldType.minuteOfDay()));
}
public void testWith3c() {
Partial test = createHourMinPartial();
Partial result = test.with(DateTimeFieldType.dayOfMonth(), 15);
check(test, 10, 20);
assertEquals(3, result.size());
assertEquals(true, result.isSupported(DateTimeFieldType.dayOfMonth()));
assertEquals(true, result.isSupported(DateTimeFieldType.hourOfDay()));
assertEquals(true, result.isSupported(DateTimeFieldType.minuteOfHour()));
assertEquals(DateTimeFieldType.dayOfMonth(), result.getFieldType(0));
assertEquals(DateTimeFieldType.hourOfDay(), result.getFieldType(1));
assertEquals(DateTimeFieldType.minuteOfHour(), result.getFieldType(2));
assertEquals(10, result.get(DateTimeFieldType.hourOfDay()));
assertEquals(20, result.get(DateTimeFieldType.minuteOfHour()));
assertEquals(15, result.get(DateTimeFieldType.dayOfMonth()));
}
public void testWith3d() {
Partial test = new Partial(DateTimeFieldType.year(), 2005);
Partial result = test.with(DateTimeFieldType.monthOfYear(), 6);
assertEquals(2, result.size());
assertEquals(2005, result.get(DateTimeFieldType.year()));
assertEquals(6, result.get(DateTimeFieldType.monthOfYear()));
}
public void testWith3e() {
Partial test = new Partial(DateTimeFieldType.era(), 1);
Partial result = test.with(DateTimeFieldType.halfdayOfDay(), 0);
assertEquals(2, result.size());
assertEquals(1, result.get(DateTimeFieldType.era()));
assertEquals(0, result.get(DateTimeFieldType.halfdayOfDay()));
assertEquals(0, result.indexOf(DateTimeFieldType.era()));
assertEquals(1, result.indexOf(DateTimeFieldType.halfdayOfDay()));
}
public void testWith3f() {
Partial test = new Partial(DateTimeFieldType.halfdayOfDay(), 0);
Partial result = test.with(DateTimeFieldType.era(), 1);
assertEquals(2, result.size());
assertEquals(1, result.get(DateTimeFieldType.era()));
assertEquals(0, result.get(DateTimeFieldType.halfdayOfDay()));
assertEquals(0, result.indexOf(DateTimeFieldType.era()));
assertEquals(1, result.indexOf(DateTimeFieldType.halfdayOfDay()));
}
public void testWith4() {
Partial test = createHourMinPartial();
Partial result = test.with(DateTimeFieldType.hourOfDay(), 10);
assertSame(test, result);
}
//-----------------------------------------------------------------------
public void testWithout1() {
Partial test = createHourMinPartial();
Partial result = test.without(DateTimeFieldType.year());
check(test, 10, 20);
check(result, 10, 20);
}
public void testWithout2() {
Partial test = createHourMinPartial();
Partial result = test.without((DateTimeFieldType) null);
check(test, 10, 20);
check(result, 10, 20);
}
public void testWithout3() {
Partial test = createHourMinPartial();
Partial result = test.without(DateTimeFieldType.hourOfDay());
check(test, 10, 20);
assertEquals(1, result.size());
assertEquals(false, result.isSupported(DateTimeFieldType.hourOfDay()));
assertEquals(true, result.isSupported(DateTimeFieldType.minuteOfHour()));
assertEquals(DateTimeFieldType.minuteOfHour(), result.getFieldType(0));
}
public void testWithout4() {
Partial test = createHourMinPartial();
Partial result = test.without(DateTimeFieldType.minuteOfHour());
check(test, 10, 20);
assertEquals(1, result.size());
assertEquals(true, result.isSupported(DateTimeFieldType.hourOfDay()));
assertEquals(false, result.isSupported(DateTimeFieldType.minuteOfHour()));
assertEquals(DateTimeFieldType.hourOfDay(), result.getFieldType(0));
}
public void testWithout5() {
Partial test = new Partial(DateTimeFieldType.hourOfDay(), 12);
Partial result = test.without(DateTimeFieldType.hourOfDay());
assertEquals(0, result.size());
assertEquals(false, result.isSupported(DateTimeFieldType.hourOfDay()));
}
//-----------------------------------------------------------------------
public void testWithField1() {
Partial test = createHourMinPartial();
Partial result = test.withField(DateTimeFieldType.hourOfDay(), 15);
check(test, 10, 20);
check(result, 15, 20);
}
public void testWithField2() {
Partial test = createHourMinPartial();
try {
test.withField(null, 6);
fail();
} catch (IllegalArgumentException ex) {}
check(test, 10, 20);
}
public void testWithField3() {
Partial test = createHourMinPartial();
try {
test.withField(DateTimeFieldType.dayOfMonth(), 6);
fail();
} catch (IllegalArgumentException ex) {}
check(test, 10, 20);
}
public void testWithField4() {
Partial test = createHourMinPartial();
Partial result = test.withField(DateTimeFieldType.hourOfDay(), 10);
assertSame(test, result);
}
//-----------------------------------------------------------------------
public void testWithFieldAdded1() {
Partial test = createHourMinPartial();
Partial result = test.withFieldAdded(DurationFieldType.hours(), 6);
assertEquals(createHourMinPartial(), test);
check(test, 10, 20);
check(result, 16, 20);
}
public void testWithFieldAdded2() {
Partial test = createHourMinPartial();
try {
test.withFieldAdded(null, 0);
fail();
} catch (IllegalArgumentException ex) {}
check(test, 10, 20);
}
public void testWithFieldAdded3() {
Partial test = createHourMinPartial();
try {
test.withFieldAdded(null, 6);
fail();
} catch (IllegalArgumentException ex) {}
check(test, 10, 20);
}
public void testWithFieldAdded4() {
Partial test = createHourMinPartial();
Partial result = test.withFieldAdded(DurationFieldType.hours(), 0);
assertSame(test, result);
}
public void testWithFieldAdded5() {
Partial test = createHourMinPartial();
try {
test.withFieldAdded(DurationFieldType.days(), 6);
fail();
} catch (IllegalArgumentException ex) {}
check(test, 10, 20);
}
public void testWithFieldAdded6() {
Partial test = createHourMinPartial();
try {
test.withFieldAdded(DurationFieldType.hours(), 16);
fail();
} catch (IllegalArgumentException ex) {
// expected
}
check(test, 10, 20);
}
public void testWithFieldAdded7() {
Partial test = createHourMinPartial(23, 59, ISO_UTC);
try {
test.withFieldAdded(DurationFieldType.minutes(), 1);
fail();
} catch (IllegalArgumentException ex) {
// expected
}
check(test, 23, 59);
test = createHourMinPartial(23, 59, ISO_UTC);
try {
test.withFieldAdded(DurationFieldType.hours(), 1);
fail();
} catch (IllegalArgumentException ex) {
// expected
}
check(test, 23, 59);
}
public void testWithFieldAdded8() {
Partial test = createHourMinPartial(0, 0, ISO_UTC);
try {
test.withFieldAdded(DurationFieldType.minutes(), -1);
fail();
} catch (IllegalArgumentException ex) {
// expected
}
check(test, 0, 0);
test = createHourMinPartial(0, 0, ISO_UTC);
try {
test.withFieldAdded(DurationFieldType.hours(), -1);
fail();
} catch (IllegalArgumentException ex) {
// expected
}
check(test, 0, 0);
}
//-----------------------------------------------------------------------
public void testWithFieldAddWrapped1() {
Partial test = createHourMinPartial();
Partial result = test.withFieldAddWrapped(DurationFieldType.hours(), 6);
assertEquals(createHourMinPartial(), test);
check(test, 10, 20);
check(result, 16, 20);
}
public void testWithFieldAddWrapped2() {
Partial test = createHourMinPartial();
try {
test.withFieldAddWrapped(null, 0);
fail();
} catch (IllegalArgumentException ex) {}
check(test, 10, 20);
}
public void testWithFieldAddWrapped3() {
Partial test = createHourMinPartial();
try {
test.withFieldAddWrapped(null, 6);
fail();
} catch (IllegalArgumentException ex) {}
check(test, 10, 20);
}
public void testWithFieldAddWrapped4() {
Partial test = createHourMinPartial();
Partial result = test.withFieldAddWrapped(DurationFieldType.hours(), 0);
assertSame(test, result);
}
public void testWithFieldAddWrapped5() {
Partial test = createHourMinPartial();
try {
test.withFieldAddWrapped(DurationFieldType.days(), 6);
fail();
} catch (IllegalArgumentException ex) {}
check(test, 10, 20);
}
public void testWithFieldAddWrapped6() {
Partial test = createHourMinPartial();
Partial result = test.withFieldAddWrapped(DurationFieldType.hours(), 16);
assertEquals(createHourMinPartial(), test);
check(test, 10, 20);
check(result, 2, 20);
}
public void testWithFieldAddWrapped7() {
Partial test = createHourMinPartial(23, 59, ISO_UTC);
Partial result = test.withFieldAddWrapped(DurationFieldType.minutes(), 1);
check(test, 23, 59);
check(result, 0, 0);
test = createHourMinPartial(23, 59, ISO_UTC);
result = test.withFieldAddWrapped(DurationFieldType.hours(), 1);
check(test, 23, 59);
check(result, 0, 59);
}
public void testWithFieldAddWrapped8() {
Partial test = createHourMinPartial(0, 0, ISO_UTC);
Partial result = test.withFieldAddWrapped(DurationFieldType.minutes(), -1);
check(test, 0, 0);
check(result, 23, 59);
test = createHourMinPartial(0, 0, ISO_UTC);
result = test.withFieldAddWrapped(DurationFieldType.hours(), -1);
check(test, 0, 0);
check(result, 23, 0);
}
//-----------------------------------------------------------------------
public void testPlus_RP() {
Partial test = createHourMinPartial(BUDDHIST_LONDON);
Partial result = test.plus(new Period(1, 2, 3, 4, 5, 6, 7, 8));
check(test, 10, 20);
check(result, 15, 26);
result = test.plus((ReadablePeriod) null);
assertSame(test, result);
}
//-----------------------------------------------------------------------
public void testMinus_RP() {
Partial test = createHourMinPartial(BUDDHIST_LONDON);
Partial result = test.minus(new Period(1, 1, 1, 1, 1, 1, 1, 1));
check(test, 10, 20);
check(result, 9, 19);
result = test.minus((ReadablePeriod) null);
assertSame(test, result);
}
//-----------------------------------------------------------------------
public void testToDateTime_RI() {
Partial base = createHourMinPartial(COPTIC_PARIS);
DateTime dt = new DateTime(0L); // LONDON zone
assertEquals("1970-01-01T01:00:00.000+01:00", dt.toString());
DateTime test = base.toDateTime(dt);
check(base, 10, 20);
assertEquals("1970-01-01T01:00:00.000+01:00", dt.toString());
assertEquals("1970-01-01T10:20:00.000+01:00", test.toString());
}
public void testToDateTime_nullRI() {
Partial base = createHourMinPartial(1, 2, ISO_UTC);
DateTimeUtils.setCurrentMillisFixed(TEST_TIME2);
DateTime test = base.toDateTime((ReadableInstant) null);
check(base, 1, 2);
assertEquals("1970-01-02T01:02:07.008+01:00", test.toString());
}
//-----------------------------------------------------------------------
public void testProperty() {
Partial test = createHourMinPartial();
assertNotNull(test.property(DateTimeFieldType.hourOfDay()));
assertNotNull(test.property(DateTimeFieldType.minuteOfHour()));
try {
test.property(DateTimeFieldType.secondOfDay());
fail();
} catch (IllegalArgumentException ex) {}
try {
test.property(null);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testSerialization() throws Exception {
Partial test = createHourMinPartial(COPTIC_PARIS);
ByteArrayOutputStream baos = new ByteArrayOutputStream();
ObjectOutputStream oos = new ObjectOutputStream(baos);
oos.writeObject(test);
byte[] bytes = baos.toByteArray();
oos.close();
ByteArrayInputStream bais = new ByteArrayInputStream(bytes);
ObjectInputStream ois = new ObjectInputStream(bais);
Partial result = (Partial) ois.readObject();
ois.close();
assertEquals(test, result);
assertTrue(Arrays.equals(test.getValues(), result.getValues()));
assertTrue(Arrays.equals(test.getFields(), result.getFields()));
assertEquals(test.getChronology(), result.getChronology());
}
//-----------------------------------------------------------------------
public void testGetFormatter1() {
Partial test = new Partial(DateTimeFieldType.year(), 2005);
assertEquals("2005", test.getFormatter().print(test));
test = test.with(DateTimeFieldType.monthOfYear(), 6);
assertEquals("2005-06", test.getFormatter().print(test));
test = test.with(DateTimeFieldType.dayOfMonth(), 25);
assertEquals("2005-06-25", test.getFormatter().print(test));
test = test.without(DateTimeFieldType.monthOfYear());
assertEquals("2005--25", test.getFormatter().print(test));
}
public void testGetFormatter2() {
Partial test = new Partial();
assertEquals(null, test.getFormatter());
test = test.with(DateTimeFieldType.era(), 1);
assertEquals(null, test.getFormatter());
test = test.with(DateTimeFieldType.halfdayOfDay(), 0);
assertEquals(null, test.getFormatter());
}
public void testGetFormatter3() {
Partial test = new Partial(DateTimeFieldType.dayOfWeek(), 5);
assertEquals("-W-5", test.getFormatter().print(test));
// contrast with testToString5
test = test.with(DateTimeFieldType.dayOfMonth(), 13);
assertEquals("---13", test.getFormatter().print(test));
}
//-----------------------------------------------------------------------
public void testToString1() {
Partial test = createHourMinPartial();
assertEquals("10:20", test.toString());
}
public void testToString2() {
Partial test = new Partial();
assertEquals("[]", test.toString());
}
public void testToString3() {
Partial test = new Partial(DateTimeFieldType.year(), 2005);
assertEquals("2005", test.toString());
test = test.with(DateTimeFieldType.monthOfYear(), 6);
assertEquals("2005-06", test.toString());
test = test.with(DateTimeFieldType.dayOfMonth(), 25);
assertEquals("2005-06-25", test.toString());
test = test.without(DateTimeFieldType.monthOfYear());
assertEquals("2005--25", test.toString());
}
public void testToString4() {
Partial test = new Partial(DateTimeFieldType.dayOfWeek(), 5);
assertEquals("-W-5", test.toString());
test = test.with(DateTimeFieldType.dayOfMonth(), 13);
assertEquals("[dayOfMonth=13, dayOfWeek=5]", test.toString());
}
public void testToString5() {
Partial test = new Partial(DateTimeFieldType.era(), 1);
assertEquals("[era=1]", test.toString());
test = test.with(DateTimeFieldType.halfdayOfDay(), 0);
assertEquals("[era=1, halfdayOfDay=0]", test.toString());
}
//-----------------------------------------------------------------------
public void testToString_String() {
Partial test = createHourMinPartial();
assertEquals("\ufffd\ufffd\ufffd\ufffd 10", test.toString("yyyy HH"));
assertEquals("10:20", test.toString((String) null));
}
//-----------------------------------------------------------------------
public void testToString_String_Locale() {
Partial test = createHourMinPartial();
assertEquals("10 20", test.toString("H m", Locale.ENGLISH));
assertEquals("10:20", test.toString(null, Locale.ENGLISH));
assertEquals("10 20", test.toString("H m", null));
assertEquals("10:20", test.toString(null, null));
}
//-----------------------------------------------------------------------
public void testToString_DTFormatter() {
Partial test = createHourMinPartial();
assertEquals("\ufffd\ufffd\ufffd\ufffd 10", test.toString(DateTimeFormat.forPattern("yyyy HH")));
assertEquals("10:20", test.toString((DateTimeFormatter) null));
}
//-----------------------------------------------------------------------
private Partial createHourMinPartial() {
return createHourMinPartial(ISO_UTC);
}
private Partial createHourMinPartial(Chronology chrono) {
return createHourMinPartial(10, 20, chrono);
}
private Partial createHourMinPartial2(Chronology chrono) {
return createHourMinPartial(15, 20, chrono);
}
private Partial createHourMinPartial(int hour, int min, Chronology chrono) {
return new Partial(
new DateTimeFieldType[] {DateTimeFieldType.hourOfDay(), DateTimeFieldType.minuteOfHour()},
new int[] {hour, min},
chrono);
}
private Partial createTODPartial(Chronology chrono) {
return new Partial(
new DateTimeFieldType[] {
DateTimeFieldType.hourOfDay(), DateTimeFieldType.minuteOfHour(),
DateTimeFieldType.secondOfMinute(), DateTimeFieldType.millisOfSecond()},
new int[] {10, 20, 30, 40},
chrono);
}
private void check(Partial test, int hour, int min) {
assertEquals(test.toString(), hour, test.get(DateTimeFieldType.hourOfDay()));
assertEquals(test.toString(), min, test.get(DateTimeFieldType.minuteOfHour()));
}
} | // You are a professional Java test case writer, please create a test case named `testWith3` for the issue `Time-88`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Time-88
//
// ## Issue-Title:
// Constructing invalid Partials
//
// ## Issue-Description:
// Partials can be constructed by invoking a constructor `Partial(DateTimeFieldType[], int[])` or by merging together a set of partials using `with`, each constructed by calling `Partial(DateTimeFieldType, int)`, e.g.:
//
//
//
// ```
// Partial a = new Partial(new DateTimeFieldType[] { year(), hourOfDay() }, new int[] { 1, 1});
// Partial b = new Partial(year(), 1).with(hourOfDay(), 1);
// assert(a == b);
// ```
//
// However, the above doesn't work in all cases:
//
//
//
// ```
// new Partial(new DateTimeFieldType[] { clockhourOfDay(), hourOfDay() }, new int[] { 1, 1}); // throws Types array must not contain duplicate
// new Partial(clockhourOfDay(), 1).with(hourOfDay(), 1); // #<Partial [clockhourOfDay=1, hourOfDay=1]>
// ```
//
// I suppose the Partials should not allow to be constructed in either case. Is that right?
//
//
// There's also a related issue (probably stems from the fact that the Partial is invalid):
//
//
//
// ```
// new Partial(clockhourOfDay(), 1).with(hourOfDay(), 1).isEqual(new Partial(hourOfDay() ,1).with(clockhourOfDay(), 1)) // throws objects must have matching field types
// ```
//
//
//
public void testWith3() {
| 366 | 4 | 359 | src/test/java/org/joda/time/TestPartial_Basics.java | src/test/java | ```markdown
## Issue-ID: Time-88
## Issue-Title:
Constructing invalid Partials
## Issue-Description:
Partials can be constructed by invoking a constructor `Partial(DateTimeFieldType[], int[])` or by merging together a set of partials using `with`, each constructed by calling `Partial(DateTimeFieldType, int)`, e.g.:
```
Partial a = new Partial(new DateTimeFieldType[] { year(), hourOfDay() }, new int[] { 1, 1});
Partial b = new Partial(year(), 1).with(hourOfDay(), 1);
assert(a == b);
```
However, the above doesn't work in all cases:
```
new Partial(new DateTimeFieldType[] { clockhourOfDay(), hourOfDay() }, new int[] { 1, 1}); // throws Types array must not contain duplicate
new Partial(clockhourOfDay(), 1).with(hourOfDay(), 1); // #<Partial [clockhourOfDay=1, hourOfDay=1]>
```
I suppose the Partials should not allow to be constructed in either case. Is that right?
There's also a related issue (probably stems from the fact that the Partial is invalid):
```
new Partial(clockhourOfDay(), 1).with(hourOfDay(), 1).isEqual(new Partial(hourOfDay() ,1).with(clockhourOfDay(), 1)) // throws objects must have matching field types
```
```
You are a professional Java test case writer, please create a test case named `testWith3` for the issue `Time-88`, utilizing the provided issue report information and the following function signature.
```java
public void testWith3() {
```
| 359 | [
"org.joda.time.Partial"
] | 6c8a8d3eedb48d935d89d44f9e8a4e8a4bba0faca1e6010d4b3a7fdc18930126 | public void testWith3() | // You are a professional Java test case writer, please create a test case named `testWith3` for the issue `Time-88`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Time-88
//
// ## Issue-Title:
// Constructing invalid Partials
//
// ## Issue-Description:
// Partials can be constructed by invoking a constructor `Partial(DateTimeFieldType[], int[])` or by merging together a set of partials using `with`, each constructed by calling `Partial(DateTimeFieldType, int)`, e.g.:
//
//
//
// ```
// Partial a = new Partial(new DateTimeFieldType[] { year(), hourOfDay() }, new int[] { 1, 1});
// Partial b = new Partial(year(), 1).with(hourOfDay(), 1);
// assert(a == b);
// ```
//
// However, the above doesn't work in all cases:
//
//
//
// ```
// new Partial(new DateTimeFieldType[] { clockhourOfDay(), hourOfDay() }, new int[] { 1, 1}); // throws Types array must not contain duplicate
// new Partial(clockhourOfDay(), 1).with(hourOfDay(), 1); // #<Partial [clockhourOfDay=1, hourOfDay=1]>
// ```
//
// I suppose the Partials should not allow to be constructed in either case. Is that right?
//
//
// There's also a related issue (probably stems from the fact that the Partial is invalid):
//
//
//
// ```
// new Partial(clockhourOfDay(), 1).with(hourOfDay(), 1).isEqual(new Partial(hourOfDay() ,1).with(clockhourOfDay(), 1)) // throws objects must have matching field types
// ```
//
//
//
| Time | /*
* Copyright 2001-2013 Stephen Colebourne
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.joda.time;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.util.Arrays;
import java.util.Locale;
import junit.framework.TestCase;
import junit.framework.TestSuite;
import org.joda.time.chrono.BuddhistChronology;
import org.joda.time.chrono.CopticChronology;
import org.joda.time.chrono.ISOChronology;
import org.joda.time.format.DateTimeFormat;
import org.joda.time.format.DateTimeFormatter;
/**
* This class is a Junit unit test for Partial.
*
* @author Stephen Colebourne
*/
public class TestPartial_Basics extends TestCase {
private static final DateTimeZone PARIS = DateTimeZone.forID("Europe/Paris");
private static final DateTimeZone LONDON = DateTimeZone.forID("Europe/London");
private static final DateTimeZone TOKYO = DateTimeZone.forID("Asia/Tokyo");
private static final Chronology COPTIC_PARIS = CopticChronology.getInstance(PARIS);
private static final Chronology COPTIC_TOKYO = CopticChronology.getInstance(TOKYO);
private static final Chronology COPTIC_UTC = CopticChronology.getInstanceUTC();
private static final Chronology ISO_UTC = ISOChronology.getInstanceUTC();
private static final Chronology BUDDHIST_LONDON = BuddhistChronology.getInstance(LONDON);
private static final Chronology BUDDHIST_TOKYO = BuddhistChronology.getInstance(TOKYO);
private static final Chronology BUDDHIST_UTC = BuddhistChronology.getInstanceUTC();
private long TEST_TIME_NOW =
10L * DateTimeConstants.MILLIS_PER_HOUR
+ 20L * DateTimeConstants.MILLIS_PER_MINUTE
+ 30L * DateTimeConstants.MILLIS_PER_SECOND
+ 40L;
private long TEST_TIME2 =
1L * DateTimeConstants.MILLIS_PER_DAY
+ 5L * DateTimeConstants.MILLIS_PER_HOUR
+ 6L * DateTimeConstants.MILLIS_PER_MINUTE
+ 7L * DateTimeConstants.MILLIS_PER_SECOND
+ 8L;
private DateTimeZone zone = null;
public static void main(String[] args) {
junit.textui.TestRunner.run(suite());
}
public static TestSuite suite() {
return new TestSuite(TestPartial_Basics.class);
}
public TestPartial_Basics(String name) {
super(name);
}
protected void setUp() throws Exception {
DateTimeUtils.setCurrentMillisFixed(TEST_TIME_NOW);
zone = DateTimeZone.getDefault();
DateTimeZone.setDefault(LONDON);
}
protected void tearDown() throws Exception {
DateTimeUtils.setCurrentMillisSystem();
DateTimeZone.setDefault(zone);
zone = null;
}
//-----------------------------------------------------------------------
public void testGet() {
Partial test = createHourMinPartial();
assertEquals(10, test.get(DateTimeFieldType.hourOfDay()));
assertEquals(20, test.get(DateTimeFieldType.minuteOfHour()));
try {
test.get(null);
fail();
} catch (IllegalArgumentException ex) {}
try {
test.get(DateTimeFieldType.secondOfMinute());
fail();
} catch (IllegalArgumentException ex) {}
}
public void testSize() {
Partial test = createHourMinPartial();
assertEquals(2, test.size());
}
public void testGetFieldType() {
Partial test = createHourMinPartial();
assertSame(DateTimeFieldType.hourOfDay(), test.getFieldType(0));
assertSame(DateTimeFieldType.minuteOfHour(), test.getFieldType(1));
try {
test.getFieldType(-1);
} catch (IndexOutOfBoundsException ex) {}
try {
test.getFieldType(2);
} catch (IndexOutOfBoundsException ex) {}
}
public void testGetFieldTypes() {
Partial test = createHourMinPartial();
DateTimeFieldType[] fields = test.getFieldTypes();
assertEquals(2, fields.length);
assertSame(DateTimeFieldType.hourOfDay(), fields[0]);
assertSame(DateTimeFieldType.minuteOfHour(), fields[1]);
assertNotSame(test.getFieldTypes(), test.getFieldTypes());
}
public void testGetField() {
Partial test = createHourMinPartial(COPTIC_PARIS);
assertSame(CopticChronology.getInstanceUTC().hourOfDay(), test.getField(0));
assertSame(CopticChronology.getInstanceUTC().minuteOfHour(), test.getField(1));
try {
test.getField(-1);
} catch (IndexOutOfBoundsException ex) {}
try {
test.getField(5);
} catch (IndexOutOfBoundsException ex) {}
}
public void testGetFields() {
Partial test = createHourMinPartial(COPTIC_PARIS);
DateTimeField[] fields = test.getFields();
assertEquals(2, fields.length);
assertSame(CopticChronology.getInstanceUTC().hourOfDay(), fields[0]);
assertSame(CopticChronology.getInstanceUTC().minuteOfHour(), fields[1]);
assertNotSame(test.getFields(), test.getFields());
}
public void testGetValue() {
Partial test = createHourMinPartial(COPTIC_PARIS);
assertEquals(10, test.getValue(0));
assertEquals(20, test.getValue(1));
try {
test.getValue(-1);
} catch (IndexOutOfBoundsException ex) {}
try {
test.getValue(2);
} catch (IndexOutOfBoundsException ex) {}
}
public void testGetValues() {
Partial test = createHourMinPartial(COPTIC_PARIS);
int[] values = test.getValues();
assertEquals(2, values.length);
assertEquals(10, values[0]);
assertEquals(20, values[1]);
assertNotSame(test.getValues(), test.getValues());
}
public void testIsSupported() {
Partial test = createHourMinPartial(COPTIC_PARIS);
assertEquals(true, test.isSupported(DateTimeFieldType.hourOfDay()));
assertEquals(true, test.isSupported(DateTimeFieldType.minuteOfHour()));
assertEquals(false, test.isSupported(DateTimeFieldType.secondOfMinute()));
assertEquals(false, test.isSupported(DateTimeFieldType.millisOfSecond()));
assertEquals(false, test.isSupported(DateTimeFieldType.dayOfMonth()));
}
@SuppressWarnings("deprecation")
public void testEqualsHashCode() {
Partial test1 = createHourMinPartial(COPTIC_PARIS);
Partial test2 = createHourMinPartial(COPTIC_PARIS);
assertEquals(true, test1.equals(test2));
assertEquals(true, test2.equals(test1));
assertEquals(true, test1.equals(test1));
assertEquals(true, test2.equals(test2));
assertEquals(true, test1.hashCode() == test2.hashCode());
assertEquals(true, test1.hashCode() == test1.hashCode());
assertEquals(true, test2.hashCode() == test2.hashCode());
Partial test3 = createHourMinPartial2(COPTIC_PARIS);
assertEquals(false, test1.equals(test3));
assertEquals(false, test2.equals(test3));
assertEquals(false, test3.equals(test1));
assertEquals(false, test3.equals(test2));
assertEquals(false, test1.hashCode() == test3.hashCode());
assertEquals(false, test2.hashCode() == test3.hashCode());
assertEquals(false, test1.equals("Hello"));
assertEquals(false, test1.equals(MockPartial.EMPTY_INSTANCE));
assertEquals(new TimeOfDay(10, 20, 30, 40), createTODPartial(ISO_UTC));
}
//-----------------------------------------------------------------------
@SuppressWarnings("deprecation")
public void testCompareTo() {
Partial test1 = createHourMinPartial();
Partial test1a = createHourMinPartial();
assertEquals(0, test1.compareTo(test1a));
assertEquals(0, test1a.compareTo(test1));
assertEquals(0, test1.compareTo(test1));
assertEquals(0, test1a.compareTo(test1a));
Partial test2 = createHourMinPartial2(ISO_UTC);
assertEquals(-1, test1.compareTo(test2));
assertEquals(+1, test2.compareTo(test1));
Partial test3 = createHourMinPartial2(COPTIC_UTC);
assertEquals(-1, test1.compareTo(test3));
assertEquals(+1, test3.compareTo(test1));
assertEquals(0, test3.compareTo(test2));
assertEquals(0, new TimeOfDay(10, 20, 30, 40).compareTo(createTODPartial(ISO_UTC)));
try {
test1.compareTo(null);
fail();
} catch (NullPointerException ex) {}
// try {
// test1.compareTo(new Date());
// fail();
// } catch (ClassCastException ex) {}
try {
test1.compareTo(new YearMonthDay());
fail();
} catch (ClassCastException ex) {}
try {
createTODPartial(ISO_UTC).without(DateTimeFieldType.hourOfDay()).compareTo(new YearMonthDay());
fail();
} catch (ClassCastException ex) {}
}
//-----------------------------------------------------------------------
public void testIsEqual_TOD() {
Partial test1 = createHourMinPartial();
Partial test1a = createHourMinPartial();
assertEquals(true, test1.isEqual(test1a));
assertEquals(true, test1a.isEqual(test1));
assertEquals(true, test1.isEqual(test1));
assertEquals(true, test1a.isEqual(test1a));
Partial test2 = createHourMinPartial2(ISO_UTC);
assertEquals(false, test1.isEqual(test2));
assertEquals(false, test2.isEqual(test1));
Partial test3 = createHourMinPartial2(COPTIC_UTC);
assertEquals(false, test1.isEqual(test3));
assertEquals(false, test3.isEqual(test1));
assertEquals(true, test3.isEqual(test2));
try {
createHourMinPartial().isEqual(null);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testIsBefore_TOD() {
Partial test1 = createHourMinPartial();
Partial test1a = createHourMinPartial();
assertEquals(false, test1.isBefore(test1a));
assertEquals(false, test1a.isBefore(test1));
assertEquals(false, test1.isBefore(test1));
assertEquals(false, test1a.isBefore(test1a));
Partial test2 = createHourMinPartial2(ISO_UTC);
assertEquals(true, test1.isBefore(test2));
assertEquals(false, test2.isBefore(test1));
Partial test3 = createHourMinPartial2(COPTIC_UTC);
assertEquals(true, test1.isBefore(test3));
assertEquals(false, test3.isBefore(test1));
assertEquals(false, test3.isBefore(test2));
try {
createHourMinPartial().isBefore(null);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testIsAfter_TOD() {
Partial test1 = createHourMinPartial();
Partial test1a = createHourMinPartial();
assertEquals(false, test1.isAfter(test1a));
assertEquals(false, test1a.isAfter(test1));
assertEquals(false, test1.isAfter(test1));
assertEquals(false, test1a.isAfter(test1a));
Partial test2 = createHourMinPartial2(ISO_UTC);
assertEquals(false, test1.isAfter(test2));
assertEquals(true, test2.isAfter(test1));
Partial test3 = createHourMinPartial2(COPTIC_UTC);
assertEquals(false, test1.isAfter(test3));
assertEquals(true, test3.isAfter(test1));
assertEquals(false, test3.isAfter(test2));
try {
createHourMinPartial().isAfter(null);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testWithChronologyRetainFields_Chrono() {
Partial base = createHourMinPartial(COPTIC_PARIS);
Partial test = base.withChronologyRetainFields(BUDDHIST_TOKYO);
check(base, 10, 20);
assertEquals(COPTIC_UTC, base.getChronology());
check(test, 10, 20);
assertEquals(BUDDHIST_UTC, test.getChronology());
}
public void testWithChronologyRetainFields_sameChrono() {
Partial base = createHourMinPartial(COPTIC_PARIS);
Partial test = base.withChronologyRetainFields(COPTIC_TOKYO);
assertSame(base, test);
}
public void testWithChronologyRetainFields_nullChrono() {
Partial base = createHourMinPartial(COPTIC_PARIS);
Partial test = base.withChronologyRetainFields(null);
check(base, 10, 20);
assertEquals(COPTIC_UTC, base.getChronology());
check(test, 10, 20);
assertEquals(ISO_UTC, test.getChronology());
}
//-----------------------------------------------------------------------
public void testWith1() {
Partial test = createHourMinPartial();
Partial result = test.with(DateTimeFieldType.hourOfDay(), 15);
check(test, 10, 20);
check(result, 15, 20);
}
public void testWith2() {
Partial test = createHourMinPartial();
try {
test.with(null, 6);
fail();
} catch (IllegalArgumentException ex) {}
check(test, 10, 20);
}
public void testWith3() {
Partial test = createHourMinPartial();
try {
test.with(DateTimeFieldType.clockhourOfDay(), 6);
fail();
} catch (IllegalArgumentException ex) {}
check(test, 10, 20);
}
public void testWith3a() {
Partial test = createHourMinPartial();
Partial result = test.with(DateTimeFieldType.secondOfMinute(), 15);
check(test, 10, 20);
assertEquals(3, result.size());
assertEquals(true, result.isSupported(DateTimeFieldType.hourOfDay()));
assertEquals(true, result.isSupported(DateTimeFieldType.minuteOfHour()));
assertEquals(true, result.isSupported(DateTimeFieldType.secondOfMinute()));
assertEquals(DateTimeFieldType.hourOfDay(), result.getFieldType(0));
assertEquals(DateTimeFieldType.minuteOfHour(), result.getFieldType(1));
assertEquals(DateTimeFieldType.secondOfMinute(), result.getFieldType(2));
assertEquals(10, result.get(DateTimeFieldType.hourOfDay()));
assertEquals(20, result.get(DateTimeFieldType.minuteOfHour()));
assertEquals(15, result.get(DateTimeFieldType.secondOfMinute()));
}
public void testWith3b() {
Partial test = createHourMinPartial();
Partial result = test.with(DateTimeFieldType.minuteOfDay(), 15);
check(test, 10, 20);
assertEquals(3, result.size());
assertEquals(true, result.isSupported(DateTimeFieldType.hourOfDay()));
assertEquals(true, result.isSupported(DateTimeFieldType.minuteOfDay()));
assertEquals(true, result.isSupported(DateTimeFieldType.minuteOfHour()));
assertEquals(DateTimeFieldType.hourOfDay(), result.getFieldType(0));
assertEquals(DateTimeFieldType.minuteOfDay(), result.getFieldType(1));
assertEquals(DateTimeFieldType.minuteOfHour(), result.getFieldType(2));
assertEquals(10, result.get(DateTimeFieldType.hourOfDay()));
assertEquals(20, result.get(DateTimeFieldType.minuteOfHour()));
assertEquals(15, result.get(DateTimeFieldType.minuteOfDay()));
}
public void testWith3c() {
Partial test = createHourMinPartial();
Partial result = test.with(DateTimeFieldType.dayOfMonth(), 15);
check(test, 10, 20);
assertEquals(3, result.size());
assertEquals(true, result.isSupported(DateTimeFieldType.dayOfMonth()));
assertEquals(true, result.isSupported(DateTimeFieldType.hourOfDay()));
assertEquals(true, result.isSupported(DateTimeFieldType.minuteOfHour()));
assertEquals(DateTimeFieldType.dayOfMonth(), result.getFieldType(0));
assertEquals(DateTimeFieldType.hourOfDay(), result.getFieldType(1));
assertEquals(DateTimeFieldType.minuteOfHour(), result.getFieldType(2));
assertEquals(10, result.get(DateTimeFieldType.hourOfDay()));
assertEquals(20, result.get(DateTimeFieldType.minuteOfHour()));
assertEquals(15, result.get(DateTimeFieldType.dayOfMonth()));
}
public void testWith3d() {
Partial test = new Partial(DateTimeFieldType.year(), 2005);
Partial result = test.with(DateTimeFieldType.monthOfYear(), 6);
assertEquals(2, result.size());
assertEquals(2005, result.get(DateTimeFieldType.year()));
assertEquals(6, result.get(DateTimeFieldType.monthOfYear()));
}
public void testWith3e() {
Partial test = new Partial(DateTimeFieldType.era(), 1);
Partial result = test.with(DateTimeFieldType.halfdayOfDay(), 0);
assertEquals(2, result.size());
assertEquals(1, result.get(DateTimeFieldType.era()));
assertEquals(0, result.get(DateTimeFieldType.halfdayOfDay()));
assertEquals(0, result.indexOf(DateTimeFieldType.era()));
assertEquals(1, result.indexOf(DateTimeFieldType.halfdayOfDay()));
}
public void testWith3f() {
Partial test = new Partial(DateTimeFieldType.halfdayOfDay(), 0);
Partial result = test.with(DateTimeFieldType.era(), 1);
assertEquals(2, result.size());
assertEquals(1, result.get(DateTimeFieldType.era()));
assertEquals(0, result.get(DateTimeFieldType.halfdayOfDay()));
assertEquals(0, result.indexOf(DateTimeFieldType.era()));
assertEquals(1, result.indexOf(DateTimeFieldType.halfdayOfDay()));
}
public void testWith4() {
Partial test = createHourMinPartial();
Partial result = test.with(DateTimeFieldType.hourOfDay(), 10);
assertSame(test, result);
}
//-----------------------------------------------------------------------
public void testWithout1() {
Partial test = createHourMinPartial();
Partial result = test.without(DateTimeFieldType.year());
check(test, 10, 20);
check(result, 10, 20);
}
public void testWithout2() {
Partial test = createHourMinPartial();
Partial result = test.without((DateTimeFieldType) null);
check(test, 10, 20);
check(result, 10, 20);
}
public void testWithout3() {
Partial test = createHourMinPartial();
Partial result = test.without(DateTimeFieldType.hourOfDay());
check(test, 10, 20);
assertEquals(1, result.size());
assertEquals(false, result.isSupported(DateTimeFieldType.hourOfDay()));
assertEquals(true, result.isSupported(DateTimeFieldType.minuteOfHour()));
assertEquals(DateTimeFieldType.minuteOfHour(), result.getFieldType(0));
}
public void testWithout4() {
Partial test = createHourMinPartial();
Partial result = test.without(DateTimeFieldType.minuteOfHour());
check(test, 10, 20);
assertEquals(1, result.size());
assertEquals(true, result.isSupported(DateTimeFieldType.hourOfDay()));
assertEquals(false, result.isSupported(DateTimeFieldType.minuteOfHour()));
assertEquals(DateTimeFieldType.hourOfDay(), result.getFieldType(0));
}
public void testWithout5() {
Partial test = new Partial(DateTimeFieldType.hourOfDay(), 12);
Partial result = test.without(DateTimeFieldType.hourOfDay());
assertEquals(0, result.size());
assertEquals(false, result.isSupported(DateTimeFieldType.hourOfDay()));
}
//-----------------------------------------------------------------------
public void testWithField1() {
Partial test = createHourMinPartial();
Partial result = test.withField(DateTimeFieldType.hourOfDay(), 15);
check(test, 10, 20);
check(result, 15, 20);
}
public void testWithField2() {
Partial test = createHourMinPartial();
try {
test.withField(null, 6);
fail();
} catch (IllegalArgumentException ex) {}
check(test, 10, 20);
}
public void testWithField3() {
Partial test = createHourMinPartial();
try {
test.withField(DateTimeFieldType.dayOfMonth(), 6);
fail();
} catch (IllegalArgumentException ex) {}
check(test, 10, 20);
}
public void testWithField4() {
Partial test = createHourMinPartial();
Partial result = test.withField(DateTimeFieldType.hourOfDay(), 10);
assertSame(test, result);
}
//-----------------------------------------------------------------------
public void testWithFieldAdded1() {
Partial test = createHourMinPartial();
Partial result = test.withFieldAdded(DurationFieldType.hours(), 6);
assertEquals(createHourMinPartial(), test);
check(test, 10, 20);
check(result, 16, 20);
}
public void testWithFieldAdded2() {
Partial test = createHourMinPartial();
try {
test.withFieldAdded(null, 0);
fail();
} catch (IllegalArgumentException ex) {}
check(test, 10, 20);
}
public void testWithFieldAdded3() {
Partial test = createHourMinPartial();
try {
test.withFieldAdded(null, 6);
fail();
} catch (IllegalArgumentException ex) {}
check(test, 10, 20);
}
public void testWithFieldAdded4() {
Partial test = createHourMinPartial();
Partial result = test.withFieldAdded(DurationFieldType.hours(), 0);
assertSame(test, result);
}
public void testWithFieldAdded5() {
Partial test = createHourMinPartial();
try {
test.withFieldAdded(DurationFieldType.days(), 6);
fail();
} catch (IllegalArgumentException ex) {}
check(test, 10, 20);
}
public void testWithFieldAdded6() {
Partial test = createHourMinPartial();
try {
test.withFieldAdded(DurationFieldType.hours(), 16);
fail();
} catch (IllegalArgumentException ex) {
// expected
}
check(test, 10, 20);
}
public void testWithFieldAdded7() {
Partial test = createHourMinPartial(23, 59, ISO_UTC);
try {
test.withFieldAdded(DurationFieldType.minutes(), 1);
fail();
} catch (IllegalArgumentException ex) {
// expected
}
check(test, 23, 59);
test = createHourMinPartial(23, 59, ISO_UTC);
try {
test.withFieldAdded(DurationFieldType.hours(), 1);
fail();
} catch (IllegalArgumentException ex) {
// expected
}
check(test, 23, 59);
}
public void testWithFieldAdded8() {
Partial test = createHourMinPartial(0, 0, ISO_UTC);
try {
test.withFieldAdded(DurationFieldType.minutes(), -1);
fail();
} catch (IllegalArgumentException ex) {
// expected
}
check(test, 0, 0);
test = createHourMinPartial(0, 0, ISO_UTC);
try {
test.withFieldAdded(DurationFieldType.hours(), -1);
fail();
} catch (IllegalArgumentException ex) {
// expected
}
check(test, 0, 0);
}
//-----------------------------------------------------------------------
public void testWithFieldAddWrapped1() {
Partial test = createHourMinPartial();
Partial result = test.withFieldAddWrapped(DurationFieldType.hours(), 6);
assertEquals(createHourMinPartial(), test);
check(test, 10, 20);
check(result, 16, 20);
}
public void testWithFieldAddWrapped2() {
Partial test = createHourMinPartial();
try {
test.withFieldAddWrapped(null, 0);
fail();
} catch (IllegalArgumentException ex) {}
check(test, 10, 20);
}
public void testWithFieldAddWrapped3() {
Partial test = createHourMinPartial();
try {
test.withFieldAddWrapped(null, 6);
fail();
} catch (IllegalArgumentException ex) {}
check(test, 10, 20);
}
public void testWithFieldAddWrapped4() {
Partial test = createHourMinPartial();
Partial result = test.withFieldAddWrapped(DurationFieldType.hours(), 0);
assertSame(test, result);
}
public void testWithFieldAddWrapped5() {
Partial test = createHourMinPartial();
try {
test.withFieldAddWrapped(DurationFieldType.days(), 6);
fail();
} catch (IllegalArgumentException ex) {}
check(test, 10, 20);
}
public void testWithFieldAddWrapped6() {
Partial test = createHourMinPartial();
Partial result = test.withFieldAddWrapped(DurationFieldType.hours(), 16);
assertEquals(createHourMinPartial(), test);
check(test, 10, 20);
check(result, 2, 20);
}
public void testWithFieldAddWrapped7() {
Partial test = createHourMinPartial(23, 59, ISO_UTC);
Partial result = test.withFieldAddWrapped(DurationFieldType.minutes(), 1);
check(test, 23, 59);
check(result, 0, 0);
test = createHourMinPartial(23, 59, ISO_UTC);
result = test.withFieldAddWrapped(DurationFieldType.hours(), 1);
check(test, 23, 59);
check(result, 0, 59);
}
public void testWithFieldAddWrapped8() {
Partial test = createHourMinPartial(0, 0, ISO_UTC);
Partial result = test.withFieldAddWrapped(DurationFieldType.minutes(), -1);
check(test, 0, 0);
check(result, 23, 59);
test = createHourMinPartial(0, 0, ISO_UTC);
result = test.withFieldAddWrapped(DurationFieldType.hours(), -1);
check(test, 0, 0);
check(result, 23, 0);
}
//-----------------------------------------------------------------------
public void testPlus_RP() {
Partial test = createHourMinPartial(BUDDHIST_LONDON);
Partial result = test.plus(new Period(1, 2, 3, 4, 5, 6, 7, 8));
check(test, 10, 20);
check(result, 15, 26);
result = test.plus((ReadablePeriod) null);
assertSame(test, result);
}
//-----------------------------------------------------------------------
public void testMinus_RP() {
Partial test = createHourMinPartial(BUDDHIST_LONDON);
Partial result = test.minus(new Period(1, 1, 1, 1, 1, 1, 1, 1));
check(test, 10, 20);
check(result, 9, 19);
result = test.minus((ReadablePeriod) null);
assertSame(test, result);
}
//-----------------------------------------------------------------------
public void testToDateTime_RI() {
Partial base = createHourMinPartial(COPTIC_PARIS);
DateTime dt = new DateTime(0L); // LONDON zone
assertEquals("1970-01-01T01:00:00.000+01:00", dt.toString());
DateTime test = base.toDateTime(dt);
check(base, 10, 20);
assertEquals("1970-01-01T01:00:00.000+01:00", dt.toString());
assertEquals("1970-01-01T10:20:00.000+01:00", test.toString());
}
public void testToDateTime_nullRI() {
Partial base = createHourMinPartial(1, 2, ISO_UTC);
DateTimeUtils.setCurrentMillisFixed(TEST_TIME2);
DateTime test = base.toDateTime((ReadableInstant) null);
check(base, 1, 2);
assertEquals("1970-01-02T01:02:07.008+01:00", test.toString());
}
//-----------------------------------------------------------------------
public void testProperty() {
Partial test = createHourMinPartial();
assertNotNull(test.property(DateTimeFieldType.hourOfDay()));
assertNotNull(test.property(DateTimeFieldType.minuteOfHour()));
try {
test.property(DateTimeFieldType.secondOfDay());
fail();
} catch (IllegalArgumentException ex) {}
try {
test.property(null);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testSerialization() throws Exception {
Partial test = createHourMinPartial(COPTIC_PARIS);
ByteArrayOutputStream baos = new ByteArrayOutputStream();
ObjectOutputStream oos = new ObjectOutputStream(baos);
oos.writeObject(test);
byte[] bytes = baos.toByteArray();
oos.close();
ByteArrayInputStream bais = new ByteArrayInputStream(bytes);
ObjectInputStream ois = new ObjectInputStream(bais);
Partial result = (Partial) ois.readObject();
ois.close();
assertEquals(test, result);
assertTrue(Arrays.equals(test.getValues(), result.getValues()));
assertTrue(Arrays.equals(test.getFields(), result.getFields()));
assertEquals(test.getChronology(), result.getChronology());
}
//-----------------------------------------------------------------------
public void testGetFormatter1() {
Partial test = new Partial(DateTimeFieldType.year(), 2005);
assertEquals("2005", test.getFormatter().print(test));
test = test.with(DateTimeFieldType.monthOfYear(), 6);
assertEquals("2005-06", test.getFormatter().print(test));
test = test.with(DateTimeFieldType.dayOfMonth(), 25);
assertEquals("2005-06-25", test.getFormatter().print(test));
test = test.without(DateTimeFieldType.monthOfYear());
assertEquals("2005--25", test.getFormatter().print(test));
}
public void testGetFormatter2() {
Partial test = new Partial();
assertEquals(null, test.getFormatter());
test = test.with(DateTimeFieldType.era(), 1);
assertEquals(null, test.getFormatter());
test = test.with(DateTimeFieldType.halfdayOfDay(), 0);
assertEquals(null, test.getFormatter());
}
public void testGetFormatter3() {
Partial test = new Partial(DateTimeFieldType.dayOfWeek(), 5);
assertEquals("-W-5", test.getFormatter().print(test));
// contrast with testToString5
test = test.with(DateTimeFieldType.dayOfMonth(), 13);
assertEquals("---13", test.getFormatter().print(test));
}
//-----------------------------------------------------------------------
public void testToString1() {
Partial test = createHourMinPartial();
assertEquals("10:20", test.toString());
}
public void testToString2() {
Partial test = new Partial();
assertEquals("[]", test.toString());
}
public void testToString3() {
Partial test = new Partial(DateTimeFieldType.year(), 2005);
assertEquals("2005", test.toString());
test = test.with(DateTimeFieldType.monthOfYear(), 6);
assertEquals("2005-06", test.toString());
test = test.with(DateTimeFieldType.dayOfMonth(), 25);
assertEquals("2005-06-25", test.toString());
test = test.without(DateTimeFieldType.monthOfYear());
assertEquals("2005--25", test.toString());
}
public void testToString4() {
Partial test = new Partial(DateTimeFieldType.dayOfWeek(), 5);
assertEquals("-W-5", test.toString());
test = test.with(DateTimeFieldType.dayOfMonth(), 13);
assertEquals("[dayOfMonth=13, dayOfWeek=5]", test.toString());
}
public void testToString5() {
Partial test = new Partial(DateTimeFieldType.era(), 1);
assertEquals("[era=1]", test.toString());
test = test.with(DateTimeFieldType.halfdayOfDay(), 0);
assertEquals("[era=1, halfdayOfDay=0]", test.toString());
}
//-----------------------------------------------------------------------
public void testToString_String() {
Partial test = createHourMinPartial();
assertEquals("\ufffd\ufffd\ufffd\ufffd 10", test.toString("yyyy HH"));
assertEquals("10:20", test.toString((String) null));
}
//-----------------------------------------------------------------------
public void testToString_String_Locale() {
Partial test = createHourMinPartial();
assertEquals("10 20", test.toString("H m", Locale.ENGLISH));
assertEquals("10:20", test.toString(null, Locale.ENGLISH));
assertEquals("10 20", test.toString("H m", null));
assertEquals("10:20", test.toString(null, null));
}
//-----------------------------------------------------------------------
public void testToString_DTFormatter() {
Partial test = createHourMinPartial();
assertEquals("\ufffd\ufffd\ufffd\ufffd 10", test.toString(DateTimeFormat.forPattern("yyyy HH")));
assertEquals("10:20", test.toString((DateTimeFormatter) null));
}
//-----------------------------------------------------------------------
private Partial createHourMinPartial() {
return createHourMinPartial(ISO_UTC);
}
private Partial createHourMinPartial(Chronology chrono) {
return createHourMinPartial(10, 20, chrono);
}
private Partial createHourMinPartial2(Chronology chrono) {
return createHourMinPartial(15, 20, chrono);
}
private Partial createHourMinPartial(int hour, int min, Chronology chrono) {
return new Partial(
new DateTimeFieldType[] {DateTimeFieldType.hourOfDay(), DateTimeFieldType.minuteOfHour()},
new int[] {hour, min},
chrono);
}
private Partial createTODPartial(Chronology chrono) {
return new Partial(
new DateTimeFieldType[] {
DateTimeFieldType.hourOfDay(), DateTimeFieldType.minuteOfHour(),
DateTimeFieldType.secondOfMinute(), DateTimeFieldType.millisOfSecond()},
new int[] {10, 20, 30, 40},
chrono);
}
private void check(Partial test, int hour, int min) {
assertEquals(test.toString(), hour, test.get(DateTimeFieldType.hourOfDay()));
assertEquals(test.toString(), min, test.get(DateTimeFieldType.minuteOfHour()));
}
} |
||
public void testExample() throws Exception {
ObjectMapper mapper = new ObjectMapper();
String json = mapper.writerWithDefaultPrettyPrinter()
.writeValueAsString(Arrays.asList(new AnimalAndType(AnimalType.Dog, new Dog())));
List<AnimalAndType> list = mapper.readerFor(new TypeReference<List<AnimalAndType>>() { })
.readValue(json);
assertNotNull(list);
} | com.fasterxml.jackson.databind.jsontype.ext.ExternalTypeIdWithEnum1328Test::testExample | src/test/java/com/fasterxml/jackson/databind/jsontype/ext/ExternalTypeIdWithEnum1328Test.java | 89 | src/test/java/com/fasterxml/jackson/databind/jsontype/ext/ExternalTypeIdWithEnum1328Test.java | testExample | package com.fasterxml.jackson.databind.jsontype.ext;
import java.io.IOException;
import java.util.Arrays;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.*;
import com.fasterxml.jackson.databind.annotation.JsonTypeIdResolver;
import com.fasterxml.jackson.databind.jsontype.TypeIdResolver;
public class ExternalTypeIdWithEnum1328Test extends BaseMapTest
{
public interface Animal { }
public static class Dog implements Animal {
public String dogStuff;
}
public enum AnimalType {
Dog;
}
public static class AnimalAndType {
public AnimalType type;
@JsonTypeInfo(use = JsonTypeInfo.Id.CLASS,
include = JsonTypeInfo.As.EXTERNAL_PROPERTY,
property = "type")
@JsonTypeIdResolver(AnimalResolver.class)
private Animal animal;
public AnimalAndType() { }
// problem is this annotation
@java.beans.ConstructorProperties({"type", "animal"})
public AnimalAndType(final AnimalType type, final Animal animal) {
this.type = type;
this.animal = animal;
}
}
static class AnimalResolver implements TypeIdResolver {
@Override
public void init(JavaType bt) { }
@Override
public String idFromValue(Object value) {
return null;
}
@Override
public String idFromValueAndType(Object value, Class<?> suggestedType) {
return null;
}
@Override
public String idFromBaseType() {
throw new UnsupportedOperationException("Missing action type information - Can not construct");
}
@Override
public JavaType typeFromId(DatabindContext context, String id) throws IOException {
if (AnimalType.Dog.toString().equals(id)) {
return context.constructType(Dog.class);
}
throw new IllegalArgumentException("What is a " + id);
}
@Override
public String getDescForKnownTypeIds() {
return null;
}
@Override
public JsonTypeInfo.Id getMechanism() {
return JsonTypeInfo.Id.CUSTOM;
}
}
public void testExample() throws Exception {
ObjectMapper mapper = new ObjectMapper();
String json = mapper.writerWithDefaultPrettyPrinter()
.writeValueAsString(Arrays.asList(new AnimalAndType(AnimalType.Dog, new Dog())));
List<AnimalAndType> list = mapper.readerFor(new TypeReference<List<AnimalAndType>>() { })
.readValue(json);
assertNotNull(list);
}
} | // You are a professional Java test case writer, please create a test case named `testExample` for the issue `JacksonDatabind-1328`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonDatabind-1328
//
// ## Issue-Title:
// External property polymorphic deserialization does not work with enums
//
// ## Issue-Description:
// versions: Jackson 2.8.1, Jackson-module-kotlin 2.8.1
//
//
// Attempting to deserialize a class using external\_property. In my case, the property is an Enum type with values matching the type name. Now that issue [#999](https://github.com/FasterXML/jackson-databind/issues/999) is fixed, I thought this would work, but now I'm getting a different error:
//
//
//
// ```
// Exception in thread "main" com.fasterxml.jackson.databind.JsonMappingException: Can not construct instance of enum.Invite, problem: argument type mismatch
// at [Source: {
// "kind": "CONTACT",
// "to": {
// "name": "Foo"
// }
// }; line: 6, column: 1]
// at com.fasterxml.jackson.databind.JsonMappingException.from(JsonMappingException.java:268)
// at com.fasterxml.jackson.databind.DeserializationContext.instantiationException(DeserializationContext.java:1405)
// at com.fasterxml.jackson.databind.deser.std.StdValueInstantiator.wrapAsJsonMappingException(StdValueInstantiator.java:468)
// at com.fasterxml.jackson.databind.deser.std.StdValueInstantiator.rewrapCtorProblem(StdValueInstantiator.java:487)
// at com.fasterxml.jackson.databind.deser.std.StdValueInstantiator.createFromObjectWith(StdValueInstantiator.java:276)
// at com.fasterxml.jackson.module.kotlin.KotlinValueInstantiator.createFromObjectWith(KotlinValueInstantiator.kt:30)
// at com.fasterxml.jackson.databind.deser.impl.PropertyBasedCreator.build(PropertyBasedCreator.java:135)
// at com.fasterxml.jackson.databind.deser.impl.ExternalTypeHandler.complete(ExternalTypeHandler.java:225)
// at com.fasterxml.jackson.databind.deser.BeanDeserializer.deserializeUsingPropertyBasedWithExternalTypeId(BeanDeserializer.java:937)
// at com.fasterxml.jackson.databind.deser.BeanDeserializer.deserializeWithExternalTypeId(BeanDeserializer.java:792)
// at com.fasterxml.jackson.databind.deser.BeanDeserializer.deserializeFromObject(BeanDeserializer.java:312)
// at com.fasterxml.jackson.databind.deser.BeanDeserializer.deserialize(BeanDeserializer.java:148)
// at com.fasterxml.jackson.databind.ObjectMapper._readMapAndClose(ObjectMapper.java:3789)
// at com.fasterxml.jackson.databind.ObjectMapper.readValue(ObjectMapper.java:2852)
// at enum.Reproduction_KindEnumKt.main(Reproduction-KindEnum.kt:49)
// at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
// at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62)
// at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43)
// at java.lang.reflect.Method.invoke(Method.java:498)
// at com.intellij.rt.execution.application.AppMain.main(AppMain.java:147)
// Caused by: java.lang.IllegalArgumentException: argument type mismatch
// at sun.reflect.NativeConstructorAccessorImpl.newInstance0(Native Method)
//
public void testExample() throws Exception {
| 89 | 98 | 81 | src/test/java/com/fasterxml/jackson/databind/jsontype/ext/ExternalTypeIdWithEnum1328Test.java | src/test/java | ```markdown
## Issue-ID: JacksonDatabind-1328
## Issue-Title:
External property polymorphic deserialization does not work with enums
## Issue-Description:
versions: Jackson 2.8.1, Jackson-module-kotlin 2.8.1
Attempting to deserialize a class using external\_property. In my case, the property is an Enum type with values matching the type name. Now that issue [#999](https://github.com/FasterXML/jackson-databind/issues/999) is fixed, I thought this would work, but now I'm getting a different error:
```
Exception in thread "main" com.fasterxml.jackson.databind.JsonMappingException: Can not construct instance of enum.Invite, problem: argument type mismatch
at [Source: {
"kind": "CONTACT",
"to": {
"name": "Foo"
}
}; line: 6, column: 1]
at com.fasterxml.jackson.databind.JsonMappingException.from(JsonMappingException.java:268)
at com.fasterxml.jackson.databind.DeserializationContext.instantiationException(DeserializationContext.java:1405)
at com.fasterxml.jackson.databind.deser.std.StdValueInstantiator.wrapAsJsonMappingException(StdValueInstantiator.java:468)
at com.fasterxml.jackson.databind.deser.std.StdValueInstantiator.rewrapCtorProblem(StdValueInstantiator.java:487)
at com.fasterxml.jackson.databind.deser.std.StdValueInstantiator.createFromObjectWith(StdValueInstantiator.java:276)
at com.fasterxml.jackson.module.kotlin.KotlinValueInstantiator.createFromObjectWith(KotlinValueInstantiator.kt:30)
at com.fasterxml.jackson.databind.deser.impl.PropertyBasedCreator.build(PropertyBasedCreator.java:135)
at com.fasterxml.jackson.databind.deser.impl.ExternalTypeHandler.complete(ExternalTypeHandler.java:225)
at com.fasterxml.jackson.databind.deser.BeanDeserializer.deserializeUsingPropertyBasedWithExternalTypeId(BeanDeserializer.java:937)
at com.fasterxml.jackson.databind.deser.BeanDeserializer.deserializeWithExternalTypeId(BeanDeserializer.java:792)
at com.fasterxml.jackson.databind.deser.BeanDeserializer.deserializeFromObject(BeanDeserializer.java:312)
at com.fasterxml.jackson.databind.deser.BeanDeserializer.deserialize(BeanDeserializer.java:148)
at com.fasterxml.jackson.databind.ObjectMapper._readMapAndClose(ObjectMapper.java:3789)
at com.fasterxml.jackson.databind.ObjectMapper.readValue(ObjectMapper.java:2852)
at enum.Reproduction_KindEnumKt.main(Reproduction-KindEnum.kt:49)
at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62)
at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43)
at java.lang.reflect.Method.invoke(Method.java:498)
at com.intellij.rt.execution.application.AppMain.main(AppMain.java:147)
Caused by: java.lang.IllegalArgumentException: argument type mismatch
at sun.reflect.NativeConstructorAccessorImpl.newInstance0(Native Method)
```
You are a professional Java test case writer, please create a test case named `testExample` for the issue `JacksonDatabind-1328`, utilizing the provided issue report information and the following function signature.
```java
public void testExample() throws Exception {
```
| 81 | [
"com.fasterxml.jackson.databind.deser.impl.ExternalTypeHandler"
] | 6d66486e1227041618eb36bf038d5073d00b3bcbdc937fbc75c469ae38a776de | public void testExample() throws Exception | // You are a professional Java test case writer, please create a test case named `testExample` for the issue `JacksonDatabind-1328`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonDatabind-1328
//
// ## Issue-Title:
// External property polymorphic deserialization does not work with enums
//
// ## Issue-Description:
// versions: Jackson 2.8.1, Jackson-module-kotlin 2.8.1
//
//
// Attempting to deserialize a class using external\_property. In my case, the property is an Enum type with values matching the type name. Now that issue [#999](https://github.com/FasterXML/jackson-databind/issues/999) is fixed, I thought this would work, but now I'm getting a different error:
//
//
//
// ```
// Exception in thread "main" com.fasterxml.jackson.databind.JsonMappingException: Can not construct instance of enum.Invite, problem: argument type mismatch
// at [Source: {
// "kind": "CONTACT",
// "to": {
// "name": "Foo"
// }
// }; line: 6, column: 1]
// at com.fasterxml.jackson.databind.JsonMappingException.from(JsonMappingException.java:268)
// at com.fasterxml.jackson.databind.DeserializationContext.instantiationException(DeserializationContext.java:1405)
// at com.fasterxml.jackson.databind.deser.std.StdValueInstantiator.wrapAsJsonMappingException(StdValueInstantiator.java:468)
// at com.fasterxml.jackson.databind.deser.std.StdValueInstantiator.rewrapCtorProblem(StdValueInstantiator.java:487)
// at com.fasterxml.jackson.databind.deser.std.StdValueInstantiator.createFromObjectWith(StdValueInstantiator.java:276)
// at com.fasterxml.jackson.module.kotlin.KotlinValueInstantiator.createFromObjectWith(KotlinValueInstantiator.kt:30)
// at com.fasterxml.jackson.databind.deser.impl.PropertyBasedCreator.build(PropertyBasedCreator.java:135)
// at com.fasterxml.jackson.databind.deser.impl.ExternalTypeHandler.complete(ExternalTypeHandler.java:225)
// at com.fasterxml.jackson.databind.deser.BeanDeserializer.deserializeUsingPropertyBasedWithExternalTypeId(BeanDeserializer.java:937)
// at com.fasterxml.jackson.databind.deser.BeanDeserializer.deserializeWithExternalTypeId(BeanDeserializer.java:792)
// at com.fasterxml.jackson.databind.deser.BeanDeserializer.deserializeFromObject(BeanDeserializer.java:312)
// at com.fasterxml.jackson.databind.deser.BeanDeserializer.deserialize(BeanDeserializer.java:148)
// at com.fasterxml.jackson.databind.ObjectMapper._readMapAndClose(ObjectMapper.java:3789)
// at com.fasterxml.jackson.databind.ObjectMapper.readValue(ObjectMapper.java:2852)
// at enum.Reproduction_KindEnumKt.main(Reproduction-KindEnum.kt:49)
// at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
// at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62)
// at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43)
// at java.lang.reflect.Method.invoke(Method.java:498)
// at com.intellij.rt.execution.application.AppMain.main(AppMain.java:147)
// Caused by: java.lang.IllegalArgumentException: argument type mismatch
// at sun.reflect.NativeConstructorAccessorImpl.newInstance0(Native Method)
//
| JacksonDatabind | package com.fasterxml.jackson.databind.jsontype.ext;
import java.io.IOException;
import java.util.Arrays;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.*;
import com.fasterxml.jackson.databind.annotation.JsonTypeIdResolver;
import com.fasterxml.jackson.databind.jsontype.TypeIdResolver;
public class ExternalTypeIdWithEnum1328Test extends BaseMapTest
{
public interface Animal { }
public static class Dog implements Animal {
public String dogStuff;
}
public enum AnimalType {
Dog;
}
public static class AnimalAndType {
public AnimalType type;
@JsonTypeInfo(use = JsonTypeInfo.Id.CLASS,
include = JsonTypeInfo.As.EXTERNAL_PROPERTY,
property = "type")
@JsonTypeIdResolver(AnimalResolver.class)
private Animal animal;
public AnimalAndType() { }
// problem is this annotation
@java.beans.ConstructorProperties({"type", "animal"})
public AnimalAndType(final AnimalType type, final Animal animal) {
this.type = type;
this.animal = animal;
}
}
static class AnimalResolver implements TypeIdResolver {
@Override
public void init(JavaType bt) { }
@Override
public String idFromValue(Object value) {
return null;
}
@Override
public String idFromValueAndType(Object value, Class<?> suggestedType) {
return null;
}
@Override
public String idFromBaseType() {
throw new UnsupportedOperationException("Missing action type information - Can not construct");
}
@Override
public JavaType typeFromId(DatabindContext context, String id) throws IOException {
if (AnimalType.Dog.toString().equals(id)) {
return context.constructType(Dog.class);
}
throw new IllegalArgumentException("What is a " + id);
}
@Override
public String getDescForKnownTypeIds() {
return null;
}
@Override
public JsonTypeInfo.Id getMechanism() {
return JsonTypeInfo.Id.CUSTOM;
}
}
public void testExample() throws Exception {
ObjectMapper mapper = new ObjectMapper();
String json = mapper.writerWithDefaultPrettyPrinter()
.writeValueAsString(Arrays.asList(new AnimalAndType(AnimalType.Dog, new Dog())));
List<AnimalAndType> list = mapper.readerFor(new TypeReference<List<AnimalAndType>>() { })
.readValue(json);
assertNotNull(list);
}
} |
||
@Test(expected = UnsupportedOperationException.class)
public void testIterator() {
final ArrayList<Chromosome> chromosomes = new ArrayList<Chromosome>();
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
final ListPopulation population = new ListPopulation(10) {
public Population nextGeneration() {
// not important
return null;
}
};
population.addChromosomes(chromosomes);
final Iterator<Chromosome> iter = population.iterator();
while (iter.hasNext()) {
iter.next();
iter.remove();
}
} | org.apache.commons.math3.genetics.ListPopulationTest::testIterator | src/test/java/org/apache/commons/math3/genetics/ListPopulationTest.java | 187 | src/test/java/org/apache/commons/math3/genetics/ListPopulationTest.java | testIterator | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.math3.genetics;
import java.util.ArrayList;
import java.util.Iterator;
import org.apache.commons.math3.exception.NotPositiveException;
import org.apache.commons.math3.exception.NumberIsTooLargeException;
import org.apache.commons.math3.exception.NumberIsTooSmallException;
import org.junit.Assert;
import org.junit.Test;
public class ListPopulationTest {
@Test
public void testGetFittestChromosome() {
Chromosome c1 = new Chromosome() {
public double fitness() {
return 0;
}
};
Chromosome c2 = new Chromosome() {
public double fitness() {
return 10;
}
};
Chromosome c3 = new Chromosome() {
public double fitness() {
return 15;
}
};
ArrayList<Chromosome> chromosomes = new ArrayList<Chromosome> ();
chromosomes.add(c1);
chromosomes.add(c2);
chromosomes.add(c3);
ListPopulation population = new ListPopulation(chromosomes, 10) {
public Population nextGeneration() {
// not important
return null;
}
};
Assert.assertEquals(c3, population.getFittestChromosome());
}
@Test
public void testChromosomes() {
final ArrayList<Chromosome> chromosomes = new ArrayList<Chromosome> ();
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
final ListPopulation population = new ListPopulation(10) {
public Population nextGeneration() {
// not important
return null;
}
};
population.addChromosomes(chromosomes);
Assert.assertEquals(chromosomes, population.getChromosomes());
Assert.assertEquals(chromosomes.toString(), population.toString());
population.setPopulationLimit(50);
Assert.assertEquals(50, population.getPopulationLimit());
}
@Test(expected = NotPositiveException.class)
public void testSetPopulationLimit() {
final ListPopulation population = new ListPopulation(10) {
public Population nextGeneration() {
// not important
return null;
}
};
population.setPopulationLimit(-50);
}
@Test(expected = NotPositiveException.class)
public void testConstructorPopulationLimitNotPositive() {
new ListPopulation(-10) {
public Population nextGeneration() {
// not important
return null;
}
};
}
@Test(expected = NotPositiveException.class)
public void testChromosomeListConstructorPopulationLimitNotPositive() {
final ArrayList<Chromosome> chromosomes = new ArrayList<Chromosome> ();
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
new ListPopulation(chromosomes, -10) {
public Population nextGeneration() {
// not important
return null;
}
};
}
@Test(expected = NumberIsTooLargeException.class)
public void testConstructorListOfChromosomesBiggerThanPopulationSize() {
final ArrayList<Chromosome> chromosomes = new ArrayList<Chromosome> ();
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
new ListPopulation(chromosomes, 1) {
public Population nextGeneration() {
// not important
return null;
}
};
}
@Test(expected=NumberIsTooLargeException.class)
public void testAddTooManyChromosomes() {
final ArrayList<Chromosome> chromosomes = new ArrayList<Chromosome> ();
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
final ListPopulation population = new ListPopulation(2) {
public Population nextGeneration() {
// not important
return null;
}
};
population.addChromosomes(chromosomes);
}
@Test(expected=NumberIsTooLargeException.class)
public void testAddTooManyChromosomesSingleCall() {
final ListPopulation population = new ListPopulation(2) {
public Population nextGeneration() {
// not important
return null;
}
};
for (int i = 0; i <= population.getPopulationLimit(); i++) {
population.addChromosome(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
}
}
@Test(expected = UnsupportedOperationException.class)
public void testIterator() {
final ArrayList<Chromosome> chromosomes = new ArrayList<Chromosome>();
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
final ListPopulation population = new ListPopulation(10) {
public Population nextGeneration() {
// not important
return null;
}
};
population.addChromosomes(chromosomes);
final Iterator<Chromosome> iter = population.iterator();
while (iter.hasNext()) {
iter.next();
iter.remove();
}
}
@Test(expected=NumberIsTooSmallException.class)
public void testSetPopulationLimitTooSmall() {
final ArrayList<Chromosome> chromosomes = new ArrayList<Chromosome> ();
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
final ListPopulation population = new ListPopulation(chromosomes, 3) {
public Population nextGeneration() {
// not important
return null;
}
};
population.setPopulationLimit(2);
}
} | // You are a professional Java test case writer, please create a test case named `testIterator` for the issue `Math-MATH-779`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Math-MATH-779
//
// ## Issue-Title:
// ListPopulation Iterator allows you to remove chromosomes from the population.
//
// ## Issue-Description:
//
// Calling the iterator method of ListPopulation returns an iterator of the protected modifiable list. Before returning the iterator we should wrap it in an unmodifiable list.
//
//
//
//
//
@Test(expected = UnsupportedOperationException.class)
public void testIterator() {
| 187 | 34 | 166 | src/test/java/org/apache/commons/math3/genetics/ListPopulationTest.java | src/test/java | ```markdown
## Issue-ID: Math-MATH-779
## Issue-Title:
ListPopulation Iterator allows you to remove chromosomes from the population.
## Issue-Description:
Calling the iterator method of ListPopulation returns an iterator of the protected modifiable list. Before returning the iterator we should wrap it in an unmodifiable list.
```
You are a professional Java test case writer, please create a test case named `testIterator` for the issue `Math-MATH-779`, utilizing the provided issue report information and the following function signature.
```java
@Test(expected = UnsupportedOperationException.class)
public void testIterator() {
```
| 166 | [
"org.apache.commons.math3.genetics.ListPopulation"
] | 6e1eda59635ac5fbb79e3e8849345b824061ee9c4cbf831ad1df670bb6f06170 | @Test(expected = UnsupportedOperationException.class)
public void testIterator() | // You are a professional Java test case writer, please create a test case named `testIterator` for the issue `Math-MATH-779`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Math-MATH-779
//
// ## Issue-Title:
// ListPopulation Iterator allows you to remove chromosomes from the population.
//
// ## Issue-Description:
//
// Calling the iterator method of ListPopulation returns an iterator of the protected modifiable list. Before returning the iterator we should wrap it in an unmodifiable list.
//
//
//
//
//
| Math | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.math3.genetics;
import java.util.ArrayList;
import java.util.Iterator;
import org.apache.commons.math3.exception.NotPositiveException;
import org.apache.commons.math3.exception.NumberIsTooLargeException;
import org.apache.commons.math3.exception.NumberIsTooSmallException;
import org.junit.Assert;
import org.junit.Test;
public class ListPopulationTest {
@Test
public void testGetFittestChromosome() {
Chromosome c1 = new Chromosome() {
public double fitness() {
return 0;
}
};
Chromosome c2 = new Chromosome() {
public double fitness() {
return 10;
}
};
Chromosome c3 = new Chromosome() {
public double fitness() {
return 15;
}
};
ArrayList<Chromosome> chromosomes = new ArrayList<Chromosome> ();
chromosomes.add(c1);
chromosomes.add(c2);
chromosomes.add(c3);
ListPopulation population = new ListPopulation(chromosomes, 10) {
public Population nextGeneration() {
// not important
return null;
}
};
Assert.assertEquals(c3, population.getFittestChromosome());
}
@Test
public void testChromosomes() {
final ArrayList<Chromosome> chromosomes = new ArrayList<Chromosome> ();
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
final ListPopulation population = new ListPopulation(10) {
public Population nextGeneration() {
// not important
return null;
}
};
population.addChromosomes(chromosomes);
Assert.assertEquals(chromosomes, population.getChromosomes());
Assert.assertEquals(chromosomes.toString(), population.toString());
population.setPopulationLimit(50);
Assert.assertEquals(50, population.getPopulationLimit());
}
@Test(expected = NotPositiveException.class)
public void testSetPopulationLimit() {
final ListPopulation population = new ListPopulation(10) {
public Population nextGeneration() {
// not important
return null;
}
};
population.setPopulationLimit(-50);
}
@Test(expected = NotPositiveException.class)
public void testConstructorPopulationLimitNotPositive() {
new ListPopulation(-10) {
public Population nextGeneration() {
// not important
return null;
}
};
}
@Test(expected = NotPositiveException.class)
public void testChromosomeListConstructorPopulationLimitNotPositive() {
final ArrayList<Chromosome> chromosomes = new ArrayList<Chromosome> ();
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
new ListPopulation(chromosomes, -10) {
public Population nextGeneration() {
// not important
return null;
}
};
}
@Test(expected = NumberIsTooLargeException.class)
public void testConstructorListOfChromosomesBiggerThanPopulationSize() {
final ArrayList<Chromosome> chromosomes = new ArrayList<Chromosome> ();
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
new ListPopulation(chromosomes, 1) {
public Population nextGeneration() {
// not important
return null;
}
};
}
@Test(expected=NumberIsTooLargeException.class)
public void testAddTooManyChromosomes() {
final ArrayList<Chromosome> chromosomes = new ArrayList<Chromosome> ();
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
final ListPopulation population = new ListPopulation(2) {
public Population nextGeneration() {
// not important
return null;
}
};
population.addChromosomes(chromosomes);
}
@Test(expected=NumberIsTooLargeException.class)
public void testAddTooManyChromosomesSingleCall() {
final ListPopulation population = new ListPopulation(2) {
public Population nextGeneration() {
// not important
return null;
}
};
for (int i = 0; i <= population.getPopulationLimit(); i++) {
population.addChromosome(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
}
}
@Test(expected = UnsupportedOperationException.class)
public void testIterator() {
final ArrayList<Chromosome> chromosomes = new ArrayList<Chromosome>();
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
final ListPopulation population = new ListPopulation(10) {
public Population nextGeneration() {
// not important
return null;
}
};
population.addChromosomes(chromosomes);
final Iterator<Chromosome> iter = population.iterator();
while (iter.hasNext()) {
iter.next();
iter.remove();
}
}
@Test(expected=NumberIsTooSmallException.class)
public void testSetPopulationLimitTooSmall() {
final ArrayList<Chromosome> chromosomes = new ArrayList<Chromosome> ();
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
chromosomes.add(new DummyBinaryChromosome(BinaryChromosome.randomBinaryRepresentation(3)));
final ListPopulation population = new ListPopulation(chromosomes, 3) {
public Population nextGeneration() {
// not important
return null;
}
};
population.setPopulationLimit(2);
}
} |
||
public void testSupplementaryUnescaping() {
NumericEntityUnescaper neu = new NumericEntityUnescaper();
String input = "𐰢";
String expected = "\uD803\uDC22";
String result = neu.translate(input);
assertEquals("Failed to unescape numeric entities supplementary characters", expected, result);
} | org.apache.commons.lang3.text.translate.NumericEntityUnescaperTest::testSupplementaryUnescaping | src/test/java/org/apache/commons/lang3/text/translate/NumericEntityUnescaperTest.java | 33 | src/test/java/org/apache/commons/lang3/text/translate/NumericEntityUnescaperTest.java | testSupplementaryUnescaping | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.lang3.text.translate;
import junit.framework.TestCase;
/**
* Unit tests for {@link org.apache.commons.lang3.text.translate.NumericEntityUnescaper}.
*/
public class NumericEntityUnescaperTest extends TestCase {
public void testSupplementaryUnescaping() {
NumericEntityUnescaper neu = new NumericEntityUnescaper();
String input = "𐰢";
String expected = "\uD803\uDC22";
String result = neu.translate(input);
assertEquals("Failed to unescape numeric entities supplementary characters", expected, result);
}
} | // You are a professional Java test case writer, please create a test case named `testSupplementaryUnescaping` for the issue `Lang-LANG-617`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Lang-LANG-617
//
// ## Issue-Title:
// StringEscapeUtils.escapeXML() can't process UTF-16 supplementary characters
//
// ## Issue-Description:
//
// Supplementary characters in UTF-16 are those whose code points are above 0xffff, that is, require more than 1 Java char to be encoded, as explained here: <http://java.sun.com/developer/technicalArticles/Intl/Supplementary/>
//
//
// Currently, StringEscapeUtils.escapeXML() isn't aware of this coding scheme and treats each char as one character, which is not always right.
//
//
// A possible solution in class Entities would be:
//
//
// public void escape(Writer writer, String str) throws IOException {
//
// int len = str.length();
//
// for (int i = 0; i < len; i++) {
//
// int code = str.codePointAt;
//
// String entityName = this.entityName(code);
//
// if (entityName != null)
//
//
// {
// writer.write('&');
// writer.write(entityName);
// writer.write(';');
// }
// else if (code > 0x7F)
//
//
// {
// writer.write("&#");
// writer.write(code);
// writer.write(';');
// }
// else
//
//
// {
// writer.write((char) code);
// }
//
// if (code > 0xffff)
//
//
// {
// i++;
// }
// }
//
// }
//
//
// Besides fixing escapeXML(), this will also affect HTML escaping functions. I guess that's a good thing, but please remember I have only tested escapeXML().
//
//
//
//
//
public void testSupplementaryUnescaping() {
| 33 | 28 | 26 | src/test/java/org/apache/commons/lang3/text/translate/NumericEntityUnescaperTest.java | src/test/java | ```markdown
## Issue-ID: Lang-LANG-617
## Issue-Title:
StringEscapeUtils.escapeXML() can't process UTF-16 supplementary characters
## Issue-Description:
Supplementary characters in UTF-16 are those whose code points are above 0xffff, that is, require more than 1 Java char to be encoded, as explained here: <http://java.sun.com/developer/technicalArticles/Intl/Supplementary/>
Currently, StringEscapeUtils.escapeXML() isn't aware of this coding scheme and treats each char as one character, which is not always right.
A possible solution in class Entities would be:
public void escape(Writer writer, String str) throws IOException {
int len = str.length();
for (int i = 0; i < len; i++) {
int code = str.codePointAt;
String entityName = this.entityName(code);
if (entityName != null)
{
writer.write('&');
writer.write(entityName);
writer.write(';');
}
else if (code > 0x7F)
{
writer.write("&#");
writer.write(code);
writer.write(';');
}
else
{
writer.write((char) code);
}
if (code > 0xffff)
{
i++;
}
}
}
Besides fixing escapeXML(), this will also affect HTML escaping functions. I guess that's a good thing, but please remember I have only tested escapeXML().
```
You are a professional Java test case writer, please create a test case named `testSupplementaryUnescaping` for the issue `Lang-LANG-617`, utilizing the provided issue report information and the following function signature.
```java
public void testSupplementaryUnescaping() {
```
| 26 | [
"org.apache.commons.lang3.text.translate.NumericEntityUnescaper"
] | 6ed8ed22d585c11cee2ef619deca5bf764360855bbfdc0bfb6214beaccd12a11 | public void testSupplementaryUnescaping() | // You are a professional Java test case writer, please create a test case named `testSupplementaryUnescaping` for the issue `Lang-LANG-617`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Lang-LANG-617
//
// ## Issue-Title:
// StringEscapeUtils.escapeXML() can't process UTF-16 supplementary characters
//
// ## Issue-Description:
//
// Supplementary characters in UTF-16 are those whose code points are above 0xffff, that is, require more than 1 Java char to be encoded, as explained here: <http://java.sun.com/developer/technicalArticles/Intl/Supplementary/>
//
//
// Currently, StringEscapeUtils.escapeXML() isn't aware of this coding scheme and treats each char as one character, which is not always right.
//
//
// A possible solution in class Entities would be:
//
//
// public void escape(Writer writer, String str) throws IOException {
//
// int len = str.length();
//
// for (int i = 0; i < len; i++) {
//
// int code = str.codePointAt;
//
// String entityName = this.entityName(code);
//
// if (entityName != null)
//
//
// {
// writer.write('&');
// writer.write(entityName);
// writer.write(';');
// }
// else if (code > 0x7F)
//
//
// {
// writer.write("&#");
// writer.write(code);
// writer.write(';');
// }
// else
//
//
// {
// writer.write((char) code);
// }
//
// if (code > 0xffff)
//
//
// {
// i++;
// }
// }
//
// }
//
//
// Besides fixing escapeXML(), this will also affect HTML escaping functions. I guess that's a good thing, but please remember I have only tested escapeXML().
//
//
//
//
//
| Lang | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.lang3.text.translate;
import junit.framework.TestCase;
/**
* Unit tests for {@link org.apache.commons.lang3.text.translate.NumericEntityUnescaper}.
*/
public class NumericEntityUnescaperTest extends TestCase {
public void testSupplementaryUnescaping() {
NumericEntityUnescaper neu = new NumericEntityUnescaper();
String input = "𐰢";
String expected = "\uD803\uDC22";
String result = neu.translate(input);
assertEquals("Failed to unescape numeric entities supplementary characters", expected, result);
}
} |
||
public void testRootEndpoints() throws Exception {
UnivariateRealFunction f = new SinFunction();
UnivariateRealSolver solver = new BrentSolver(f);
// endpoint is root
double result = solver.solve(Math.PI, 4);
assertEquals(result, Math.PI, solver.getAbsoluteAccuracy());
result = solver.solve(3, Math.PI);
assertEquals(result, Math.PI, solver.getAbsoluteAccuracy());
} | org.apache.commons.math.analysis.BrentSolverTest::testRootEndpoints | src/test/org/apache/commons/math/analysis/BrentSolverTest.java | 280 | src/test/org/apache/commons/math/analysis/BrentSolverTest.java | testRootEndpoints | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.math.analysis;
import org.apache.commons.math.MathException;
import junit.framework.Test;
import junit.framework.TestCase;
import junit.framework.TestSuite;
/**
* Testcase for UnivariateRealSolver.
* Because Brent-Dekker is guaranteed to converge in less than the default
* maximum iteration count due to bisection fallback, it is quite hard to
* debug. I include measured iteration counts plus one in order to detect
* regressions. On average Brent-Dekker should use 4..5 iterations for the
* default absolute accuracy of 10E-8 for sinus and the quintic function around
* zero, and 5..10 iterations for the other zeros.
*
* @version $Revision:670469 $ $Date:2008-06-23 10:01:38 +0200 (lun., 23 juin 2008) $
*/
public final class BrentSolverTest extends TestCase {
public BrentSolverTest(String name) {
super(name);
}
public static Test suite() {
TestSuite suite = new TestSuite(BrentSolverTest.class);
suite.setName("UnivariateRealSolver Tests");
return suite;
}
public void testSinZero() throws MathException {
// The sinus function is behaved well around the root at #pi. The second
// order derivative is zero, which means linar approximating methods will
// still converge quadratically.
UnivariateRealFunction f = new SinFunction();
double result;
UnivariateRealSolver solver = new BrentSolver(f);
// Somewhat benign interval. The function is monotone.
result = solver.solve(3, 4);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, Math.PI, solver.getAbsoluteAccuracy());
// 4 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 5);
// Larger and somewhat less benign interval. The function is grows first.
result = solver.solve(1, 4);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, Math.PI, solver.getAbsoluteAccuracy());
// 5 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 6);
solver = new SecantSolver(f);
result = solver.solve(3, 4);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, Math.PI, solver.getAbsoluteAccuracy());
// 4 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 5);
result = solver.solve(1, 4);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, Math.PI, solver.getAbsoluteAccuracy());
// 5 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 6);
assertEquals(result, solver.getResult(), 0);
}
public void testQuinticZero() throws MathException {
// The quintic function has zeros at 0, +-0.5 and +-1.
// Around the root of 0 the function is well behaved, with a second derivative
// of zero a 0.
// The other roots are less well to find, in particular the root at 1, because
// the function grows fast for x>1.
// The function has extrema (first derivative is zero) at 0.27195613 and 0.82221643,
// intervals containing these values are harder for the solvers.
UnivariateRealFunction f = new QuinticFunction();
double result;
// Brent-Dekker solver.
UnivariateRealSolver solver = new BrentSolver(f);
// Symmetric bracket around 0. Test whether solvers can handle hitting
// the root in the first iteration.
result = solver.solve(-0.2, 0.2);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 0, solver.getAbsoluteAccuracy());
assertTrue(solver.getIterationCount() <= 2);
// 1 iterations on i586 JDK 1.4.1.
// Asymmetric bracket around 0, just for fun. Contains extremum.
result = solver.solve(-0.1, 0.3);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 0, solver.getAbsoluteAccuracy());
// 5 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 6);
// Large bracket around 0. Contains two extrema.
result = solver.solve(-0.3, 0.45);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 0, solver.getAbsoluteAccuracy());
// 6 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 7);
// Benign bracket around 0.5, function is monotonous.
result = solver.solve(0.3, 0.7);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 0.5, solver.getAbsoluteAccuracy());
// 6 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 7);
// Less benign bracket around 0.5, contains one extremum.
result = solver.solve(0.2, 0.6);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 0.5, solver.getAbsoluteAccuracy());
// 6 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 7);
// Large, less benign bracket around 0.5, contains both extrema.
result = solver.solve(0.05, 0.95);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 0.5, solver.getAbsoluteAccuracy());
// 8 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 9);
// Relatively benign bracket around 1, function is monotonous. Fast growth for x>1
// is still a problem.
result = solver.solve(0.85, 1.25);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 1.0, solver.getAbsoluteAccuracy());
// 8 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 9);
// Less benign bracket around 1 with extremum.
result = solver.solve(0.8, 1.2);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 1.0, solver.getAbsoluteAccuracy());
// 8 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 9);
// Large bracket around 1. Monotonous.
result = solver.solve(0.85, 1.75);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 1.0, solver.getAbsoluteAccuracy());
// 10 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 11);
// Large bracket around 1. Interval contains extremum.
result = solver.solve(0.55, 1.45);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 1.0, solver.getAbsoluteAccuracy());
// 7 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 8);
// Very large bracket around 1 for testing fast growth behaviour.
result = solver.solve(0.85, 5);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 1.0, solver.getAbsoluteAccuracy());
// 12 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 13);
// Secant solver.
solver = new SecantSolver(f);
result = solver.solve(-0.2, 0.2);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 0, solver.getAbsoluteAccuracy());
// 1 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 2);
result = solver.solve(-0.1, 0.3);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 0, solver.getAbsoluteAccuracy());
// 5 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 6);
result = solver.solve(-0.3, 0.45);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 0, solver.getAbsoluteAccuracy());
// 6 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 7);
result = solver.solve(0.3, 0.7);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 0.5, solver.getAbsoluteAccuracy());
// 7 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 8);
result = solver.solve(0.2, 0.6);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 0.5, solver.getAbsoluteAccuracy());
// 6 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 7);
result = solver.solve(0.05, 0.95);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 0.5, solver.getAbsoluteAccuracy());
// 8 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 9);
result = solver.solve(0.85, 1.25);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 1.0, solver.getAbsoluteAccuracy());
// 10 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 11);
result = solver.solve(0.8, 1.2);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 1.0, solver.getAbsoluteAccuracy());
// 8 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 9);
result = solver.solve(0.85, 1.75);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 1.0, solver.getAbsoluteAccuracy());
// 14 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 15);
// The followig is especially slow because the solver first has to reduce
// the bracket to exclude the extremum. After that, convergence is rapide.
result = solver.solve(0.55, 1.45);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 1.0, solver.getAbsoluteAccuracy());
// 7 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 8);
result = solver.solve(0.85, 5);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 1.0, solver.getAbsoluteAccuracy());
// 14 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 15);
// Static solve method
result = UnivariateRealSolverUtils.solve(f, -0.2, 0.2);
assertEquals(result, 0, solver.getAbsoluteAccuracy());
result = UnivariateRealSolverUtils.solve(f, -0.1, 0.3);
assertEquals(result, 0, 1E-8);
result = UnivariateRealSolverUtils.solve(f, -0.3, 0.45);
assertEquals(result, 0, 1E-6);
result = UnivariateRealSolverUtils.solve(f, 0.3, 0.7);
assertEquals(result, 0.5, 1E-6);
result = UnivariateRealSolverUtils.solve(f, 0.2, 0.6);
assertEquals(result, 0.5, 1E-6);
result = UnivariateRealSolverUtils.solve(f, 0.05, 0.95);
assertEquals(result, 0.5, 1E-6);
result = UnivariateRealSolverUtils.solve(f, 0.85, 1.25);
assertEquals(result, 1.0, 1E-6);
result = UnivariateRealSolverUtils.solve(f, 0.8, 1.2);
assertEquals(result, 1.0, 1E-6);
result = UnivariateRealSolverUtils.solve(f, 0.85, 1.75);
assertEquals(result, 1.0, 1E-6);
result = UnivariateRealSolverUtils.solve(f, 0.55, 1.45);
assertEquals(result, 1.0, 1E-6);
result = UnivariateRealSolverUtils.solve(f, 0.85, 5);
assertEquals(result, 1.0, 1E-6);
}
public void testRootEndpoints() throws Exception {
UnivariateRealFunction f = new SinFunction();
UnivariateRealSolver solver = new BrentSolver(f);
// endpoint is root
double result = solver.solve(Math.PI, 4);
assertEquals(result, Math.PI, solver.getAbsoluteAccuracy());
result = solver.solve(3, Math.PI);
assertEquals(result, Math.PI, solver.getAbsoluteAccuracy());
}
public void testBadEndpoints() throws Exception {
UnivariateRealFunction f = new SinFunction();
UnivariateRealSolver solver = new BrentSolver(f);
try { // bad interval
solver.solve(1, -1);
fail("Expecting IllegalArgumentException - bad interval");
} catch (IllegalArgumentException ex) {
// expected
}
try { // no bracket
solver.solve(1, 1.5);
fail("Expecting IllegalArgumentException - non-bracketing");
} catch (IllegalArgumentException ex) {
// expected
}
}
public void testInitialGuess() throws MathException {
MonitoredFunction f = new MonitoredFunction(new QuinticFunction());
UnivariateRealSolver solver = new BrentSolver(f);
double result;
// no guess
result = solver.solve(0.6, 7.0);
assertEquals(result, 1.0, solver.getAbsoluteAccuracy());
int referenceCallsCount = f.getCallsCount();
assertTrue(referenceCallsCount >= 13);
// invalid guess (it *is* a root, but outside of the range)
try {
result = solver.solve(0.6, 7.0, 0.0);
fail("an IllegalArgumentException was expected");
} catch (IllegalArgumentException iae) {
// expected behaviour
} catch (Exception e) {
fail("wrong exception caught: " + e.getMessage());
}
// bad guess
f.setCallsCount(0);
result = solver.solve(0.6, 7.0, 0.61);
assertEquals(result, 1.0, solver.getAbsoluteAccuracy());
assertTrue(f.getCallsCount() > referenceCallsCount);
// good guess
f.setCallsCount(0);
result = solver.solve(0.6, 7.0, 0.999999);
assertEquals(result, 1.0, solver.getAbsoluteAccuracy());
assertTrue(f.getCallsCount() < referenceCallsCount);
// perfect guess
f.setCallsCount(0);
result = solver.solve(0.6, 7.0, 1.0);
assertEquals(result, 1.0, solver.getAbsoluteAccuracy());
assertEquals(0, solver.getIterationCount());
assertEquals(1, f.getCallsCount());
}
} | // You are a professional Java test case writer, please create a test case named `testRootEndpoints` for the issue `Math-MATH-204`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Math-MATH-204
//
// ## Issue-Title:
// BrentSolver throws IllegalArgumentException
//
// ## Issue-Description:
//
// I am getting this exception:
//
//
// java.lang.IllegalArgumentException: Function values at endpoints do not have different signs. Endpoints: [-100000.0,1.7976931348623157E308] Values: [0.0,-101945.04630982173]
//
// at org.apache.commons.math.analysis.BrentSolver.solve(BrentSolver.java:99)
//
// at org.apache.commons.math.analysis.BrentSolver.solve(BrentSolver.java:62)
//
//
// The exception should not be thrown with values [0.0,-101945.04630982173] because 0.0 is positive.
//
// According to Brent Worden, the algorithm should stop and return 0 as the root instead of throwing an exception.
//
//
// The problem comes from this method:
//
// public double solve(double min, double max) throws MaxIterationsExceededException,
//
// FunctionEvaluationException {
//
//
// clearResult();
//
// verifyInterval(min, max);
//
//
// double yMin = f.value(min);
//
// double yMax = f.value(max);
//
//
// // Verify bracketing
//
// if (yMin \* yMax >= 0)
//
//
// {
// throw new IllegalArgumentException
// ("Function values at endpoints do not have different signs." +
// " Endpoints: [" + min + "," + max + "]" +
// " Values: [" + yMin + "," + yMax + "]");
// }
//
// // solve using only the first endpoint as initial guess
//
// return solve(min, yMin, max, yMax, min, yMin);
//
//
// }
//
//
// One way to fix it would be to add this code after the assignment of yMin and yMax:
//
// if (yMin ==0 || yMax == 0)
//
//
// {
// return 0;
// }
//
//
//
public void testRootEndpoints() throws Exception {
| 280 | 97 | 270 | src/test/org/apache/commons/math/analysis/BrentSolverTest.java | src/test | ```markdown
## Issue-ID: Math-MATH-204
## Issue-Title:
BrentSolver throws IllegalArgumentException
## Issue-Description:
I am getting this exception:
java.lang.IllegalArgumentException: Function values at endpoints do not have different signs. Endpoints: [-100000.0,1.7976931348623157E308] Values: [0.0,-101945.04630982173]
at org.apache.commons.math.analysis.BrentSolver.solve(BrentSolver.java:99)
at org.apache.commons.math.analysis.BrentSolver.solve(BrentSolver.java:62)
The exception should not be thrown with values [0.0,-101945.04630982173] because 0.0 is positive.
According to Brent Worden, the algorithm should stop and return 0 as the root instead of throwing an exception.
The problem comes from this method:
public double solve(double min, double max) throws MaxIterationsExceededException,
FunctionEvaluationException {
clearResult();
verifyInterval(min, max);
double yMin = f.value(min);
double yMax = f.value(max);
// Verify bracketing
if (yMin \* yMax >= 0)
{
throw new IllegalArgumentException
("Function values at endpoints do not have different signs." +
" Endpoints: [" + min + "," + max + "]" +
" Values: [" + yMin + "," + yMax + "]");
}
// solve using only the first endpoint as initial guess
return solve(min, yMin, max, yMax, min, yMin);
}
One way to fix it would be to add this code after the assignment of yMin and yMax:
if (yMin ==0 || yMax == 0)
{
return 0;
}
```
You are a professional Java test case writer, please create a test case named `testRootEndpoints` for the issue `Math-MATH-204`, utilizing the provided issue report information and the following function signature.
```java
public void testRootEndpoints() throws Exception {
```
| 270 | [
"org.apache.commons.math.analysis.BrentSolver"
] | 6f731e5d2a9eb10ab8a1a30ad3a9e1e1098006c54de22fa3fc099dc96735be3b | public void testRootEndpoints() throws Exception | // You are a professional Java test case writer, please create a test case named `testRootEndpoints` for the issue `Math-MATH-204`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Math-MATH-204
//
// ## Issue-Title:
// BrentSolver throws IllegalArgumentException
//
// ## Issue-Description:
//
// I am getting this exception:
//
//
// java.lang.IllegalArgumentException: Function values at endpoints do not have different signs. Endpoints: [-100000.0,1.7976931348623157E308] Values: [0.0,-101945.04630982173]
//
// at org.apache.commons.math.analysis.BrentSolver.solve(BrentSolver.java:99)
//
// at org.apache.commons.math.analysis.BrentSolver.solve(BrentSolver.java:62)
//
//
// The exception should not be thrown with values [0.0,-101945.04630982173] because 0.0 is positive.
//
// According to Brent Worden, the algorithm should stop and return 0 as the root instead of throwing an exception.
//
//
// The problem comes from this method:
//
// public double solve(double min, double max) throws MaxIterationsExceededException,
//
// FunctionEvaluationException {
//
//
// clearResult();
//
// verifyInterval(min, max);
//
//
// double yMin = f.value(min);
//
// double yMax = f.value(max);
//
//
// // Verify bracketing
//
// if (yMin \* yMax >= 0)
//
//
// {
// throw new IllegalArgumentException
// ("Function values at endpoints do not have different signs." +
// " Endpoints: [" + min + "," + max + "]" +
// " Values: [" + yMin + "," + yMax + "]");
// }
//
// // solve using only the first endpoint as initial guess
//
// return solve(min, yMin, max, yMax, min, yMin);
//
//
// }
//
//
// One way to fix it would be to add this code after the assignment of yMin and yMax:
//
// if (yMin ==0 || yMax == 0)
//
//
// {
// return 0;
// }
//
//
//
| Math | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.math.analysis;
import org.apache.commons.math.MathException;
import junit.framework.Test;
import junit.framework.TestCase;
import junit.framework.TestSuite;
/**
* Testcase for UnivariateRealSolver.
* Because Brent-Dekker is guaranteed to converge in less than the default
* maximum iteration count due to bisection fallback, it is quite hard to
* debug. I include measured iteration counts plus one in order to detect
* regressions. On average Brent-Dekker should use 4..5 iterations for the
* default absolute accuracy of 10E-8 for sinus and the quintic function around
* zero, and 5..10 iterations for the other zeros.
*
* @version $Revision:670469 $ $Date:2008-06-23 10:01:38 +0200 (lun., 23 juin 2008) $
*/
public final class BrentSolverTest extends TestCase {
public BrentSolverTest(String name) {
super(name);
}
public static Test suite() {
TestSuite suite = new TestSuite(BrentSolverTest.class);
suite.setName("UnivariateRealSolver Tests");
return suite;
}
public void testSinZero() throws MathException {
// The sinus function is behaved well around the root at #pi. The second
// order derivative is zero, which means linar approximating methods will
// still converge quadratically.
UnivariateRealFunction f = new SinFunction();
double result;
UnivariateRealSolver solver = new BrentSolver(f);
// Somewhat benign interval. The function is monotone.
result = solver.solve(3, 4);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, Math.PI, solver.getAbsoluteAccuracy());
// 4 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 5);
// Larger and somewhat less benign interval. The function is grows first.
result = solver.solve(1, 4);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, Math.PI, solver.getAbsoluteAccuracy());
// 5 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 6);
solver = new SecantSolver(f);
result = solver.solve(3, 4);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, Math.PI, solver.getAbsoluteAccuracy());
// 4 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 5);
result = solver.solve(1, 4);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, Math.PI, solver.getAbsoluteAccuracy());
// 5 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 6);
assertEquals(result, solver.getResult(), 0);
}
public void testQuinticZero() throws MathException {
// The quintic function has zeros at 0, +-0.5 and +-1.
// Around the root of 0 the function is well behaved, with a second derivative
// of zero a 0.
// The other roots are less well to find, in particular the root at 1, because
// the function grows fast for x>1.
// The function has extrema (first derivative is zero) at 0.27195613 and 0.82221643,
// intervals containing these values are harder for the solvers.
UnivariateRealFunction f = new QuinticFunction();
double result;
// Brent-Dekker solver.
UnivariateRealSolver solver = new BrentSolver(f);
// Symmetric bracket around 0. Test whether solvers can handle hitting
// the root in the first iteration.
result = solver.solve(-0.2, 0.2);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 0, solver.getAbsoluteAccuracy());
assertTrue(solver.getIterationCount() <= 2);
// 1 iterations on i586 JDK 1.4.1.
// Asymmetric bracket around 0, just for fun. Contains extremum.
result = solver.solve(-0.1, 0.3);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 0, solver.getAbsoluteAccuracy());
// 5 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 6);
// Large bracket around 0. Contains two extrema.
result = solver.solve(-0.3, 0.45);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 0, solver.getAbsoluteAccuracy());
// 6 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 7);
// Benign bracket around 0.5, function is monotonous.
result = solver.solve(0.3, 0.7);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 0.5, solver.getAbsoluteAccuracy());
// 6 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 7);
// Less benign bracket around 0.5, contains one extremum.
result = solver.solve(0.2, 0.6);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 0.5, solver.getAbsoluteAccuracy());
// 6 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 7);
// Large, less benign bracket around 0.5, contains both extrema.
result = solver.solve(0.05, 0.95);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 0.5, solver.getAbsoluteAccuracy());
// 8 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 9);
// Relatively benign bracket around 1, function is monotonous. Fast growth for x>1
// is still a problem.
result = solver.solve(0.85, 1.25);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 1.0, solver.getAbsoluteAccuracy());
// 8 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 9);
// Less benign bracket around 1 with extremum.
result = solver.solve(0.8, 1.2);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 1.0, solver.getAbsoluteAccuracy());
// 8 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 9);
// Large bracket around 1. Monotonous.
result = solver.solve(0.85, 1.75);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 1.0, solver.getAbsoluteAccuracy());
// 10 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 11);
// Large bracket around 1. Interval contains extremum.
result = solver.solve(0.55, 1.45);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 1.0, solver.getAbsoluteAccuracy());
// 7 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 8);
// Very large bracket around 1 for testing fast growth behaviour.
result = solver.solve(0.85, 5);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 1.0, solver.getAbsoluteAccuracy());
// 12 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 13);
// Secant solver.
solver = new SecantSolver(f);
result = solver.solve(-0.2, 0.2);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 0, solver.getAbsoluteAccuracy());
// 1 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 2);
result = solver.solve(-0.1, 0.3);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 0, solver.getAbsoluteAccuracy());
// 5 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 6);
result = solver.solve(-0.3, 0.45);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 0, solver.getAbsoluteAccuracy());
// 6 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 7);
result = solver.solve(0.3, 0.7);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 0.5, solver.getAbsoluteAccuracy());
// 7 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 8);
result = solver.solve(0.2, 0.6);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 0.5, solver.getAbsoluteAccuracy());
// 6 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 7);
result = solver.solve(0.05, 0.95);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 0.5, solver.getAbsoluteAccuracy());
// 8 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 9);
result = solver.solve(0.85, 1.25);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 1.0, solver.getAbsoluteAccuracy());
// 10 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 11);
result = solver.solve(0.8, 1.2);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 1.0, solver.getAbsoluteAccuracy());
// 8 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 9);
result = solver.solve(0.85, 1.75);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 1.0, solver.getAbsoluteAccuracy());
// 14 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 15);
// The followig is especially slow because the solver first has to reduce
// the bracket to exclude the extremum. After that, convergence is rapide.
result = solver.solve(0.55, 1.45);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 1.0, solver.getAbsoluteAccuracy());
// 7 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 8);
result = solver.solve(0.85, 5);
//System.out.println(
// "Root: " + result + " Iterations: " + solver.getIterationCount());
assertEquals(result, 1.0, solver.getAbsoluteAccuracy());
// 14 iterations on i586 JDK 1.4.1.
assertTrue(solver.getIterationCount() <= 15);
// Static solve method
result = UnivariateRealSolverUtils.solve(f, -0.2, 0.2);
assertEquals(result, 0, solver.getAbsoluteAccuracy());
result = UnivariateRealSolverUtils.solve(f, -0.1, 0.3);
assertEquals(result, 0, 1E-8);
result = UnivariateRealSolverUtils.solve(f, -0.3, 0.45);
assertEquals(result, 0, 1E-6);
result = UnivariateRealSolverUtils.solve(f, 0.3, 0.7);
assertEquals(result, 0.5, 1E-6);
result = UnivariateRealSolverUtils.solve(f, 0.2, 0.6);
assertEquals(result, 0.5, 1E-6);
result = UnivariateRealSolverUtils.solve(f, 0.05, 0.95);
assertEquals(result, 0.5, 1E-6);
result = UnivariateRealSolverUtils.solve(f, 0.85, 1.25);
assertEquals(result, 1.0, 1E-6);
result = UnivariateRealSolverUtils.solve(f, 0.8, 1.2);
assertEquals(result, 1.0, 1E-6);
result = UnivariateRealSolverUtils.solve(f, 0.85, 1.75);
assertEquals(result, 1.0, 1E-6);
result = UnivariateRealSolverUtils.solve(f, 0.55, 1.45);
assertEquals(result, 1.0, 1E-6);
result = UnivariateRealSolverUtils.solve(f, 0.85, 5);
assertEquals(result, 1.0, 1E-6);
}
public void testRootEndpoints() throws Exception {
UnivariateRealFunction f = new SinFunction();
UnivariateRealSolver solver = new BrentSolver(f);
// endpoint is root
double result = solver.solve(Math.PI, 4);
assertEquals(result, Math.PI, solver.getAbsoluteAccuracy());
result = solver.solve(3, Math.PI);
assertEquals(result, Math.PI, solver.getAbsoluteAccuracy());
}
public void testBadEndpoints() throws Exception {
UnivariateRealFunction f = new SinFunction();
UnivariateRealSolver solver = new BrentSolver(f);
try { // bad interval
solver.solve(1, -1);
fail("Expecting IllegalArgumentException - bad interval");
} catch (IllegalArgumentException ex) {
// expected
}
try { // no bracket
solver.solve(1, 1.5);
fail("Expecting IllegalArgumentException - non-bracketing");
} catch (IllegalArgumentException ex) {
// expected
}
}
public void testInitialGuess() throws MathException {
MonitoredFunction f = new MonitoredFunction(new QuinticFunction());
UnivariateRealSolver solver = new BrentSolver(f);
double result;
// no guess
result = solver.solve(0.6, 7.0);
assertEquals(result, 1.0, solver.getAbsoluteAccuracy());
int referenceCallsCount = f.getCallsCount();
assertTrue(referenceCallsCount >= 13);
// invalid guess (it *is* a root, but outside of the range)
try {
result = solver.solve(0.6, 7.0, 0.0);
fail("an IllegalArgumentException was expected");
} catch (IllegalArgumentException iae) {
// expected behaviour
} catch (Exception e) {
fail("wrong exception caught: " + e.getMessage());
}
// bad guess
f.setCallsCount(0);
result = solver.solve(0.6, 7.0, 0.61);
assertEquals(result, 1.0, solver.getAbsoluteAccuracy());
assertTrue(f.getCallsCount() > referenceCallsCount);
// good guess
f.setCallsCount(0);
result = solver.solve(0.6, 7.0, 0.999999);
assertEquals(result, 1.0, solver.getAbsoluteAccuracy());
assertTrue(f.getCallsCount() < referenceCallsCount);
// perfect guess
f.setCallsCount(0);
result = solver.solve(0.6, 7.0, 1.0);
assertEquals(result, 1.0, solver.getAbsoluteAccuracy());
assertEquals(0, solver.getIterationCount());
assertEquals(1, f.getCallsCount());
}
} |
||
public void testAbbreviate() {
// check null and empty are returned respectively
assertNull(WordUtils.abbreviate(null, 1,-1,""));
assertEquals(StringUtils.EMPTY, WordUtils.abbreviate("", 1,-1,""));
// test upper limit
assertEquals("01234", WordUtils.abbreviate("0123456789", 0,5,""));
assertEquals("01234", WordUtils.abbreviate("0123456789", 5, 2,""));
assertEquals("012", WordUtils.abbreviate("012 3456789", 2, 5,""));
assertEquals("012 3", WordUtils.abbreviate("012 3456789", 5, 2,""));
assertEquals("0123456789", WordUtils.abbreviate("0123456789", 0,-1,""));
// test upper limit + append string
assertEquals("01234-", WordUtils.abbreviate("0123456789", 0,5,"-"));
assertEquals("01234-", WordUtils.abbreviate("0123456789", 5, 2,"-"));
assertEquals("012", WordUtils.abbreviate("012 3456789", 2, 5, null));
assertEquals("012 3", WordUtils.abbreviate("012 3456789", 5, 2,""));
assertEquals("0123456789", WordUtils.abbreviate("0123456789", 0,-1,""));
// test lower value
assertEquals("012", WordUtils.abbreviate("012 3456789", 0,5, null));
assertEquals("01234", WordUtils.abbreviate("01234 56789", 5, 10, null));
assertEquals("01 23 45 67", WordUtils.abbreviate("01 23 45 67 89", 9, -1, null));
assertEquals("01 23 45 6", WordUtils.abbreviate("01 23 45 67 89", 9, 10, null));
assertEquals("0123456789", WordUtils.abbreviate("0123456789", 15, 20, null));
// test lower value + append
assertEquals("012", WordUtils.abbreviate("012 3456789", 0,5, null));
assertEquals("01234-", WordUtils.abbreviate("01234 56789", 5, 10, "-"));
assertEquals("01 23 45 67abc", WordUtils.abbreviate("01 23 45 67 89", 9, -1, "abc"));
assertEquals("01 23 45 6", WordUtils.abbreviate("01 23 45 67 89", 9, 10, ""));
// others
assertEquals("", WordUtils.abbreviate("0123456790", 0,0,""));
assertEquals("", WordUtils.abbreviate(" 0123456790", 0,-1,""));
} | org.apache.commons.lang.WordUtilsTest::testAbbreviate | src/test/org/apache/commons/lang/WordUtilsTest.java | 400 | src/test/org/apache/commons/lang/WordUtilsTest.java | testAbbreviate | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.lang;
import java.lang.reflect.Constructor;
import java.lang.reflect.Modifier;
import junit.framework.Test;
import junit.framework.TestCase;
import junit.framework.TestSuite;
/**
* Unit tests for WordUtils class.
*
* @author <a href="mailto:[email protected]">Ringo De Smet</a>
* @author Stephen Colebourne
* @version $Id$
*/
public class WordUtilsTest extends TestCase {
public WordUtilsTest(String name) {
super(name);
}
public static Test suite() {
TestSuite suite = new TestSuite(WordUtilsTest.class);
suite.setName("WordUtilsTests");
return suite;
}
//-----------------------------------------------------------------------
public void testConstructor() {
assertNotNull(new WordUtils());
Constructor[] cons = WordUtils.class.getDeclaredConstructors();
assertEquals(1, cons.length);
assertEquals(true, Modifier.isPublic(cons[0].getModifiers()));
assertEquals(true, Modifier.isPublic(WordUtils.class.getModifiers()));
assertEquals(false, Modifier.isFinal(WordUtils.class.getModifiers()));
}
//-----------------------------------------------------------------------
public void testWrap_StringInt() {
assertEquals(null, WordUtils.wrap(null, 20));
assertEquals(null, WordUtils.wrap(null, -1));
assertEquals("", WordUtils.wrap("", 20));
assertEquals("", WordUtils.wrap("", -1));
// normal
String systemNewLine = System.getProperty("line.separator");
String input = "Here is one line of text that is going to be wrapped after 20 columns.";
String expected = "Here is one line of" + systemNewLine + "text that is going"
+ systemNewLine + "to be wrapped after" + systemNewLine + "20 columns.";
assertEquals(expected, WordUtils.wrap(input, 20));
// long word at end
input = "Click here to jump to the jakarta website - http://jakarta.apache.org";
expected = "Click here to jump" + systemNewLine + "to the jakarta" + systemNewLine
+ "website -" + systemNewLine + "http://jakarta.apache.org";
assertEquals(expected, WordUtils.wrap(input, 20));
// long word in middle
input = "Click here, http://jakarta.apache.org, to jump to the jakarta website";
expected = "Click here," + systemNewLine + "http://jakarta.apache.org," + systemNewLine
+ "to jump to the" + systemNewLine + "jakarta website";
assertEquals(expected, WordUtils.wrap(input, 20));
}
public void testWrap_StringIntStringBoolean() {
assertEquals(null, WordUtils.wrap(null, 20, "\n", false));
assertEquals(null, WordUtils.wrap(null, 20, "\n", true));
assertEquals(null, WordUtils.wrap(null, 20, null, true));
assertEquals(null, WordUtils.wrap(null, 20, null, false));
assertEquals(null, WordUtils.wrap(null, -1, null, true));
assertEquals(null, WordUtils.wrap(null, -1, null, false));
assertEquals("", WordUtils.wrap("", 20, "\n", false));
assertEquals("", WordUtils.wrap("", 20, "\n", true));
assertEquals("", WordUtils.wrap("", 20, null, false));
assertEquals("", WordUtils.wrap("", 20, null, true));
assertEquals("", WordUtils.wrap("", -1, null, false));
assertEquals("", WordUtils.wrap("", -1, null, true));
// normal
String input = "Here is one line of text that is going to be wrapped after 20 columns.";
String expected = "Here is one line of\ntext that is going\nto be wrapped after\n20 columns.";
assertEquals(expected, WordUtils.wrap(input, 20, "\n", false));
assertEquals(expected, WordUtils.wrap(input, 20, "\n", true));
// unusual newline char
input = "Here is one line of text that is going to be wrapped after 20 columns.";
expected = "Here is one line of<br />text that is going<br />to be wrapped after<br />20 columns.";
assertEquals(expected, WordUtils.wrap(input, 20, "<br />", false));
assertEquals(expected, WordUtils.wrap(input, 20, "<br />", true));
// short line length
input = "Here is one line";
expected = "Here\nis one\nline";
assertEquals(expected, WordUtils.wrap(input, 6, "\n", false));
expected = "Here\nis\none\nline";
assertEquals(expected, WordUtils.wrap(input, 2, "\n", false));
assertEquals(expected, WordUtils.wrap(input, -1, "\n", false));
// system newline char
String systemNewLine = System.getProperty("line.separator");
input = "Here is one line of text that is going to be wrapped after 20 columns.";
expected = "Here is one line of" + systemNewLine + "text that is going" + systemNewLine
+ "to be wrapped after" + systemNewLine + "20 columns.";
assertEquals(expected, WordUtils.wrap(input, 20, null, false));
assertEquals(expected, WordUtils.wrap(input, 20, null, true));
// with extra spaces
input = " Here: is one line of text that is going to be wrapped after 20 columns.";
expected = "Here: is one line\nof text that is \ngoing to be \nwrapped after 20 \ncolumns.";
assertEquals(expected, WordUtils.wrap(input, 20, "\n", false));
assertEquals(expected, WordUtils.wrap(input, 20, "\n", true));
// with tab
input = "Here is\tone line of text that is going to be wrapped after 20 columns.";
expected = "Here is\tone line of\ntext that is going\nto be wrapped after\n20 columns.";
assertEquals(expected, WordUtils.wrap(input, 20, "\n", false));
assertEquals(expected, WordUtils.wrap(input, 20, "\n", true));
// with tab at wrapColumn
input = "Here is one line of\ttext that is going to be wrapped after 20 columns.";
expected = "Here is one line\nof\ttext that is\ngoing to be wrapped\nafter 20 columns.";
assertEquals(expected, WordUtils.wrap(input, 20, "\n", false));
assertEquals(expected, WordUtils.wrap(input, 20, "\n", true));
// difference because of long word
input = "Click here to jump to the jakarta website - http://jakarta.apache.org";
expected = "Click here to jump\nto the jakarta\nwebsite -\nhttp://jakarta.apache.org";
assertEquals(expected, WordUtils.wrap(input, 20, "\n", false));
expected = "Click here to jump\nto the jakarta\nwebsite -\nhttp://jakarta.apach\ne.org";
assertEquals(expected, WordUtils.wrap(input, 20, "\n", true));
// difference because of long word in middle
input = "Click here, http://jakarta.apache.org, to jump to the jakarta website";
expected = "Click here,\nhttp://jakarta.apache.org,\nto jump to the\njakarta website";
assertEquals(expected, WordUtils.wrap(input, 20, "\n", false));
expected = "Click here,\nhttp://jakarta.apach\ne.org, to jump to\nthe jakarta website";
assertEquals(expected, WordUtils.wrap(input, 20, "\n", true));
// System.err.println(expected);
// System.err.println(WordUtils.wrap(input, 20, "\n", false));
}
//-----------------------------------------------------------------------
public void testCapitalize_String() {
assertEquals(null, WordUtils.capitalize(null));
assertEquals("", WordUtils.capitalize(""));
assertEquals(" ", WordUtils.capitalize(" "));
assertEquals("I", WordUtils.capitalize("I") );
assertEquals("I", WordUtils.capitalize("i") );
assertEquals("I Am Here 123", WordUtils.capitalize("i am here 123") );
assertEquals("I Am Here 123", WordUtils.capitalize("I Am Here 123") );
assertEquals("I Am HERE 123", WordUtils.capitalize("i am HERE 123") );
assertEquals("I AM HERE 123", WordUtils.capitalize("I AM HERE 123") );
}
public void testCapitalizeWithDelimiters_String() {
assertEquals(null, WordUtils.capitalize(null, null));
assertEquals("", WordUtils.capitalize("", new char[0]));
assertEquals(" ", WordUtils.capitalize(" ", new char[0]));
char[] chars = new char[] { '-', '+', ' ', '@' };
assertEquals("I", WordUtils.capitalize("I", chars) );
assertEquals("I", WordUtils.capitalize("i", chars) );
assertEquals("I-Am Here+123", WordUtils.capitalize("i-am here+123", chars) );
assertEquals("I Am+Here-123", WordUtils.capitalize("I Am+Here-123", chars) );
assertEquals("I+Am-HERE 123", WordUtils.capitalize("i+am-HERE 123", chars) );
assertEquals("I-AM HERE+123", WordUtils.capitalize("I-AM HERE+123", chars) );
chars = new char[] {'.'};
assertEquals("I aM.Fine", WordUtils.capitalize("i aM.fine", chars) );
assertEquals("I Am.fine", WordUtils.capitalize("i am.fine", null) );
}
public void testCapitalizeFully_String() {
assertEquals(null, WordUtils.capitalizeFully(null));
assertEquals("", WordUtils.capitalizeFully(""));
assertEquals(" ", WordUtils.capitalizeFully(" "));
assertEquals("I", WordUtils.capitalizeFully("I") );
assertEquals("I", WordUtils.capitalizeFully("i") );
assertEquals("I Am Here 123", WordUtils.capitalizeFully("i am here 123") );
assertEquals("I Am Here 123", WordUtils.capitalizeFully("I Am Here 123") );
assertEquals("I Am Here 123", WordUtils.capitalizeFully("i am HERE 123") );
assertEquals("I Am Here 123", WordUtils.capitalizeFully("I AM HERE 123") );
}
public void testCapitalizeFullyWithDelimiters_String() {
assertEquals(null, WordUtils.capitalizeFully(null, null));
assertEquals("", WordUtils.capitalizeFully("", new char[0]));
assertEquals(" ", WordUtils.capitalizeFully(" ", new char[0]));
char[] chars = new char[] { '-', '+', ' ', '@' };
assertEquals("I", WordUtils.capitalizeFully("I", chars) );
assertEquals("I", WordUtils.capitalizeFully("i", chars) );
assertEquals("I-Am Here+123", WordUtils.capitalizeFully("i-am here+123", chars) );
assertEquals("I Am+Here-123", WordUtils.capitalizeFully("I Am+Here-123", chars) );
assertEquals("I+Am-Here 123", WordUtils.capitalizeFully("i+am-HERE 123", chars) );
assertEquals("I-Am Here+123", WordUtils.capitalizeFully("I-AM HERE+123", chars) );
chars = new char[] {'.'};
assertEquals("I am.Fine", WordUtils.capitalizeFully("i aM.fine", chars) );
assertEquals("I Am.fine", WordUtils.capitalizeFully("i am.fine", null) );
}
public void testUncapitalize_String() {
assertEquals(null, WordUtils.uncapitalize(null));
assertEquals("", WordUtils.uncapitalize(""));
assertEquals(" ", WordUtils.uncapitalize(" "));
assertEquals("i", WordUtils.uncapitalize("I") );
assertEquals("i", WordUtils.uncapitalize("i") );
assertEquals("i am here 123", WordUtils.uncapitalize("i am here 123") );
assertEquals("i am here 123", WordUtils.uncapitalize("I Am Here 123") );
assertEquals("i am hERE 123", WordUtils.uncapitalize("i am HERE 123") );
assertEquals("i aM hERE 123", WordUtils.uncapitalize("I AM HERE 123") );
}
public void testUncapitalizeWithDelimiters_String() {
assertEquals(null, WordUtils.uncapitalize(null, null));
assertEquals("", WordUtils.uncapitalize("", new char[0]));
assertEquals(" ", WordUtils.uncapitalize(" ", new char[0]));
char[] chars = new char[] { '-', '+', ' ', '@' };
assertEquals("i", WordUtils.uncapitalize("I", chars) );
assertEquals("i", WordUtils.uncapitalize("i", chars) );
assertEquals("i am-here+123", WordUtils.uncapitalize("i am-here+123", chars) );
assertEquals("i+am here-123", WordUtils.uncapitalize("I+Am Here-123", chars) );
assertEquals("i-am+hERE 123", WordUtils.uncapitalize("i-am+HERE 123", chars) );
assertEquals("i aM-hERE+123", WordUtils.uncapitalize("I AM-HERE+123", chars) );
chars = new char[] {'.'};
assertEquals("i AM.fINE", WordUtils.uncapitalize("I AM.FINE", chars) );
assertEquals("i aM.FINE", WordUtils.uncapitalize("I AM.FINE", null) );
}
//-----------------------------------------------------------------------
public void testInitials_String() {
assertEquals(null, WordUtils.initials(null));
assertEquals("", WordUtils.initials(""));
assertEquals("", WordUtils.initials(" "));
assertEquals("I", WordUtils.initials("I"));
assertEquals("i", WordUtils.initials("i"));
assertEquals("BJL", WordUtils.initials("Ben John Lee"));
assertEquals("BJ", WordUtils.initials("Ben J.Lee"));
assertEquals("BJ.L", WordUtils.initials(" Ben John . Lee"));
assertEquals("iah1", WordUtils.initials("i am here 123"));
}
// -----------------------------------------------------------------------
public void testInitials_String_charArray() {
char[] array = null;
assertEquals(null, WordUtils.initials(null, array));
assertEquals("", WordUtils.initials("", array));
assertEquals("", WordUtils.initials(" ", array));
assertEquals("I", WordUtils.initials("I", array));
assertEquals("i", WordUtils.initials("i", array));
assertEquals("S", WordUtils.initials("SJC", array));
assertEquals("BJL", WordUtils.initials("Ben John Lee", array));
assertEquals("BJ", WordUtils.initials("Ben J.Lee", array));
assertEquals("BJ.L", WordUtils.initials(" Ben John . Lee", array));
assertEquals("KO", WordUtils.initials("Kay O'Murphy", array));
assertEquals("iah1", WordUtils.initials("i am here 123", array));
array = new char[0];
assertEquals(null, WordUtils.initials(null, array));
assertEquals("", WordUtils.initials("", array));
assertEquals("", WordUtils.initials(" ", array));
assertEquals("", WordUtils.initials("I", array));
assertEquals("", WordUtils.initials("i", array));
assertEquals("", WordUtils.initials("SJC", array));
assertEquals("", WordUtils.initials("Ben John Lee", array));
assertEquals("", WordUtils.initials("Ben J.Lee", array));
assertEquals("", WordUtils.initials(" Ben John . Lee", array));
assertEquals("", WordUtils.initials("Kay O'Murphy", array));
assertEquals("", WordUtils.initials("i am here 123", array));
array = " ".toCharArray();
assertEquals(null, WordUtils.initials(null, array));
assertEquals("", WordUtils.initials("", array));
assertEquals("", WordUtils.initials(" ", array));
assertEquals("I", WordUtils.initials("I", array));
assertEquals("i", WordUtils.initials("i", array));
assertEquals("S", WordUtils.initials("SJC", array));
assertEquals("BJL", WordUtils.initials("Ben John Lee", array));
assertEquals("BJ", WordUtils.initials("Ben J.Lee", array));
assertEquals("BJ.L", WordUtils.initials(" Ben John . Lee", array));
assertEquals("KO", WordUtils.initials("Kay O'Murphy", array));
assertEquals("iah1", WordUtils.initials("i am here 123", array));
array = " .".toCharArray();
assertEquals(null, WordUtils.initials(null, array));
assertEquals("", WordUtils.initials("", array));
assertEquals("", WordUtils.initials(" ", array));
assertEquals("I", WordUtils.initials("I", array));
assertEquals("i", WordUtils.initials("i", array));
assertEquals("S", WordUtils.initials("SJC", array));
assertEquals("BJL", WordUtils.initials("Ben John Lee", array));
assertEquals("BJL", WordUtils.initials("Ben J.Lee", array));
assertEquals("BJL", WordUtils.initials(" Ben John . Lee", array));
assertEquals("KO", WordUtils.initials("Kay O'Murphy", array));
assertEquals("iah1", WordUtils.initials("i am here 123", array));
array = " .'".toCharArray();
assertEquals(null, WordUtils.initials(null, array));
assertEquals("", WordUtils.initials("", array));
assertEquals("", WordUtils.initials(" ", array));
assertEquals("I", WordUtils.initials("I", array));
assertEquals("i", WordUtils.initials("i", array));
assertEquals("S", WordUtils.initials("SJC", array));
assertEquals("BJL", WordUtils.initials("Ben John Lee", array));
assertEquals("BJL", WordUtils.initials("Ben J.Lee", array));
assertEquals("BJL", WordUtils.initials(" Ben John . Lee", array));
assertEquals("KOM", WordUtils.initials("Kay O'Murphy", array));
assertEquals("iah1", WordUtils.initials("i am here 123", array));
array = "SIJo1".toCharArray();
assertEquals(null, WordUtils.initials(null, array));
assertEquals("", WordUtils.initials("", array));
assertEquals(" ", WordUtils.initials(" ", array));
assertEquals("", WordUtils.initials("I", array));
assertEquals("i", WordUtils.initials("i", array));
assertEquals("C", WordUtils.initials("SJC", array));
assertEquals("Bh", WordUtils.initials("Ben John Lee", array));
assertEquals("B.", WordUtils.initials("Ben J.Lee", array));
assertEquals(" h", WordUtils.initials(" Ben John . Lee", array));
assertEquals("K", WordUtils.initials("Kay O'Murphy", array));
assertEquals("i2", WordUtils.initials("i am here 123", array));
}
// -----------------------------------------------------------------------
public void testSwapCase_String() {
assertEquals(null, WordUtils.swapCase(null));
assertEquals("", WordUtils.swapCase(""));
assertEquals(" ", WordUtils.swapCase(" "));
assertEquals("i", WordUtils.swapCase("I") );
assertEquals("I", WordUtils.swapCase("i") );
assertEquals("I AM HERE 123", WordUtils.swapCase("i am here 123") );
assertEquals("i aM hERE 123", WordUtils.swapCase("I Am Here 123") );
assertEquals("I AM here 123", WordUtils.swapCase("i am HERE 123") );
assertEquals("i am here 123", WordUtils.swapCase("I AM HERE 123") );
String test = "This String contains a TitleCase character: \u01C8";
String expect = "tHIS sTRING CONTAINS A tITLEcASE CHARACTER: \u01C9";
assertEquals(expect, WordUtils.swapCase(test));
}
// -----------------------------------------------------------------------
public void testAbbreviate() {
// check null and empty are returned respectively
assertNull(WordUtils.abbreviate(null, 1,-1,""));
assertEquals(StringUtils.EMPTY, WordUtils.abbreviate("", 1,-1,""));
// test upper limit
assertEquals("01234", WordUtils.abbreviate("0123456789", 0,5,""));
assertEquals("01234", WordUtils.abbreviate("0123456789", 5, 2,""));
assertEquals("012", WordUtils.abbreviate("012 3456789", 2, 5,""));
assertEquals("012 3", WordUtils.abbreviate("012 3456789", 5, 2,""));
assertEquals("0123456789", WordUtils.abbreviate("0123456789", 0,-1,""));
// test upper limit + append string
assertEquals("01234-", WordUtils.abbreviate("0123456789", 0,5,"-"));
assertEquals("01234-", WordUtils.abbreviate("0123456789", 5, 2,"-"));
assertEquals("012", WordUtils.abbreviate("012 3456789", 2, 5, null));
assertEquals("012 3", WordUtils.abbreviate("012 3456789", 5, 2,""));
assertEquals("0123456789", WordUtils.abbreviate("0123456789", 0,-1,""));
// test lower value
assertEquals("012", WordUtils.abbreviate("012 3456789", 0,5, null));
assertEquals("01234", WordUtils.abbreviate("01234 56789", 5, 10, null));
assertEquals("01 23 45 67", WordUtils.abbreviate("01 23 45 67 89", 9, -1, null));
assertEquals("01 23 45 6", WordUtils.abbreviate("01 23 45 67 89", 9, 10, null));
assertEquals("0123456789", WordUtils.abbreviate("0123456789", 15, 20, null));
// test lower value + append
assertEquals("012", WordUtils.abbreviate("012 3456789", 0,5, null));
assertEquals("01234-", WordUtils.abbreviate("01234 56789", 5, 10, "-"));
assertEquals("01 23 45 67abc", WordUtils.abbreviate("01 23 45 67 89", 9, -1, "abc"));
assertEquals("01 23 45 6", WordUtils.abbreviate("01 23 45 67 89", 9, 10, ""));
// others
assertEquals("", WordUtils.abbreviate("0123456790", 0,0,""));
assertEquals("", WordUtils.abbreviate(" 0123456790", 0,-1,""));
}
} | // You are a professional Java test case writer, please create a test case named `testAbbreviate` for the issue `Lang-LANG-419`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Lang-LANG-419
//
// ## Issue-Title:
// WordUtils.abbreviate bug when lower is greater than str.length
//
// ## Issue-Description:
//
// In WordUtils.abbreviate, upper is adjusted to the length of the string, then to lower.
//
// But lower is never adjusted to the length of the string, so if lower is greater than str.lengt(), upper will be too...
//
// Then, str.substring(0, upper) throw a StringIndexOutOfBoundsException
//
//
// The fix is to adjust lower to the length of the string
//
//
//
//
//
public void testAbbreviate() {
| 400 | // ----------------------------------------------------------------------- | 45 | 365 | src/test/org/apache/commons/lang/WordUtilsTest.java | src/test | ```markdown
## Issue-ID: Lang-LANG-419
## Issue-Title:
WordUtils.abbreviate bug when lower is greater than str.length
## Issue-Description:
In WordUtils.abbreviate, upper is adjusted to the length of the string, then to lower.
But lower is never adjusted to the length of the string, so if lower is greater than str.lengt(), upper will be too...
Then, str.substring(0, upper) throw a StringIndexOutOfBoundsException
The fix is to adjust lower to the length of the string
```
You are a professional Java test case writer, please create a test case named `testAbbreviate` for the issue `Lang-LANG-419`, utilizing the provided issue report information and the following function signature.
```java
public void testAbbreviate() {
```
| 365 | [
"org.apache.commons.lang.WordUtils"
] | 70fe6488c6b2d2cc2fb2a1f51c0b2c07fe7ba83945d79ca35c9cb28de620046a | public void testAbbreviate() | // You are a professional Java test case writer, please create a test case named `testAbbreviate` for the issue `Lang-LANG-419`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Lang-LANG-419
//
// ## Issue-Title:
// WordUtils.abbreviate bug when lower is greater than str.length
//
// ## Issue-Description:
//
// In WordUtils.abbreviate, upper is adjusted to the length of the string, then to lower.
//
// But lower is never adjusted to the length of the string, so if lower is greater than str.lengt(), upper will be too...
//
// Then, str.substring(0, upper) throw a StringIndexOutOfBoundsException
//
//
// The fix is to adjust lower to the length of the string
//
//
//
//
//
| Lang | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.lang;
import java.lang.reflect.Constructor;
import java.lang.reflect.Modifier;
import junit.framework.Test;
import junit.framework.TestCase;
import junit.framework.TestSuite;
/**
* Unit tests for WordUtils class.
*
* @author <a href="mailto:[email protected]">Ringo De Smet</a>
* @author Stephen Colebourne
* @version $Id$
*/
public class WordUtilsTest extends TestCase {
public WordUtilsTest(String name) {
super(name);
}
public static Test suite() {
TestSuite suite = new TestSuite(WordUtilsTest.class);
suite.setName("WordUtilsTests");
return suite;
}
//-----------------------------------------------------------------------
public void testConstructor() {
assertNotNull(new WordUtils());
Constructor[] cons = WordUtils.class.getDeclaredConstructors();
assertEquals(1, cons.length);
assertEquals(true, Modifier.isPublic(cons[0].getModifiers()));
assertEquals(true, Modifier.isPublic(WordUtils.class.getModifiers()));
assertEquals(false, Modifier.isFinal(WordUtils.class.getModifiers()));
}
//-----------------------------------------------------------------------
public void testWrap_StringInt() {
assertEquals(null, WordUtils.wrap(null, 20));
assertEquals(null, WordUtils.wrap(null, -1));
assertEquals("", WordUtils.wrap("", 20));
assertEquals("", WordUtils.wrap("", -1));
// normal
String systemNewLine = System.getProperty("line.separator");
String input = "Here is one line of text that is going to be wrapped after 20 columns.";
String expected = "Here is one line of" + systemNewLine + "text that is going"
+ systemNewLine + "to be wrapped after" + systemNewLine + "20 columns.";
assertEquals(expected, WordUtils.wrap(input, 20));
// long word at end
input = "Click here to jump to the jakarta website - http://jakarta.apache.org";
expected = "Click here to jump" + systemNewLine + "to the jakarta" + systemNewLine
+ "website -" + systemNewLine + "http://jakarta.apache.org";
assertEquals(expected, WordUtils.wrap(input, 20));
// long word in middle
input = "Click here, http://jakarta.apache.org, to jump to the jakarta website";
expected = "Click here," + systemNewLine + "http://jakarta.apache.org," + systemNewLine
+ "to jump to the" + systemNewLine + "jakarta website";
assertEquals(expected, WordUtils.wrap(input, 20));
}
public void testWrap_StringIntStringBoolean() {
assertEquals(null, WordUtils.wrap(null, 20, "\n", false));
assertEquals(null, WordUtils.wrap(null, 20, "\n", true));
assertEquals(null, WordUtils.wrap(null, 20, null, true));
assertEquals(null, WordUtils.wrap(null, 20, null, false));
assertEquals(null, WordUtils.wrap(null, -1, null, true));
assertEquals(null, WordUtils.wrap(null, -1, null, false));
assertEquals("", WordUtils.wrap("", 20, "\n", false));
assertEquals("", WordUtils.wrap("", 20, "\n", true));
assertEquals("", WordUtils.wrap("", 20, null, false));
assertEquals("", WordUtils.wrap("", 20, null, true));
assertEquals("", WordUtils.wrap("", -1, null, false));
assertEquals("", WordUtils.wrap("", -1, null, true));
// normal
String input = "Here is one line of text that is going to be wrapped after 20 columns.";
String expected = "Here is one line of\ntext that is going\nto be wrapped after\n20 columns.";
assertEquals(expected, WordUtils.wrap(input, 20, "\n", false));
assertEquals(expected, WordUtils.wrap(input, 20, "\n", true));
// unusual newline char
input = "Here is one line of text that is going to be wrapped after 20 columns.";
expected = "Here is one line of<br />text that is going<br />to be wrapped after<br />20 columns.";
assertEquals(expected, WordUtils.wrap(input, 20, "<br />", false));
assertEquals(expected, WordUtils.wrap(input, 20, "<br />", true));
// short line length
input = "Here is one line";
expected = "Here\nis one\nline";
assertEquals(expected, WordUtils.wrap(input, 6, "\n", false));
expected = "Here\nis\none\nline";
assertEquals(expected, WordUtils.wrap(input, 2, "\n", false));
assertEquals(expected, WordUtils.wrap(input, -1, "\n", false));
// system newline char
String systemNewLine = System.getProperty("line.separator");
input = "Here is one line of text that is going to be wrapped after 20 columns.";
expected = "Here is one line of" + systemNewLine + "text that is going" + systemNewLine
+ "to be wrapped after" + systemNewLine + "20 columns.";
assertEquals(expected, WordUtils.wrap(input, 20, null, false));
assertEquals(expected, WordUtils.wrap(input, 20, null, true));
// with extra spaces
input = " Here: is one line of text that is going to be wrapped after 20 columns.";
expected = "Here: is one line\nof text that is \ngoing to be \nwrapped after 20 \ncolumns.";
assertEquals(expected, WordUtils.wrap(input, 20, "\n", false));
assertEquals(expected, WordUtils.wrap(input, 20, "\n", true));
// with tab
input = "Here is\tone line of text that is going to be wrapped after 20 columns.";
expected = "Here is\tone line of\ntext that is going\nto be wrapped after\n20 columns.";
assertEquals(expected, WordUtils.wrap(input, 20, "\n", false));
assertEquals(expected, WordUtils.wrap(input, 20, "\n", true));
// with tab at wrapColumn
input = "Here is one line of\ttext that is going to be wrapped after 20 columns.";
expected = "Here is one line\nof\ttext that is\ngoing to be wrapped\nafter 20 columns.";
assertEquals(expected, WordUtils.wrap(input, 20, "\n", false));
assertEquals(expected, WordUtils.wrap(input, 20, "\n", true));
// difference because of long word
input = "Click here to jump to the jakarta website - http://jakarta.apache.org";
expected = "Click here to jump\nto the jakarta\nwebsite -\nhttp://jakarta.apache.org";
assertEquals(expected, WordUtils.wrap(input, 20, "\n", false));
expected = "Click here to jump\nto the jakarta\nwebsite -\nhttp://jakarta.apach\ne.org";
assertEquals(expected, WordUtils.wrap(input, 20, "\n", true));
// difference because of long word in middle
input = "Click here, http://jakarta.apache.org, to jump to the jakarta website";
expected = "Click here,\nhttp://jakarta.apache.org,\nto jump to the\njakarta website";
assertEquals(expected, WordUtils.wrap(input, 20, "\n", false));
expected = "Click here,\nhttp://jakarta.apach\ne.org, to jump to\nthe jakarta website";
assertEquals(expected, WordUtils.wrap(input, 20, "\n", true));
// System.err.println(expected);
// System.err.println(WordUtils.wrap(input, 20, "\n", false));
}
//-----------------------------------------------------------------------
public void testCapitalize_String() {
assertEquals(null, WordUtils.capitalize(null));
assertEquals("", WordUtils.capitalize(""));
assertEquals(" ", WordUtils.capitalize(" "));
assertEquals("I", WordUtils.capitalize("I") );
assertEquals("I", WordUtils.capitalize("i") );
assertEquals("I Am Here 123", WordUtils.capitalize("i am here 123") );
assertEquals("I Am Here 123", WordUtils.capitalize("I Am Here 123") );
assertEquals("I Am HERE 123", WordUtils.capitalize("i am HERE 123") );
assertEquals("I AM HERE 123", WordUtils.capitalize("I AM HERE 123") );
}
public void testCapitalizeWithDelimiters_String() {
assertEquals(null, WordUtils.capitalize(null, null));
assertEquals("", WordUtils.capitalize("", new char[0]));
assertEquals(" ", WordUtils.capitalize(" ", new char[0]));
char[] chars = new char[] { '-', '+', ' ', '@' };
assertEquals("I", WordUtils.capitalize("I", chars) );
assertEquals("I", WordUtils.capitalize("i", chars) );
assertEquals("I-Am Here+123", WordUtils.capitalize("i-am here+123", chars) );
assertEquals("I Am+Here-123", WordUtils.capitalize("I Am+Here-123", chars) );
assertEquals("I+Am-HERE 123", WordUtils.capitalize("i+am-HERE 123", chars) );
assertEquals("I-AM HERE+123", WordUtils.capitalize("I-AM HERE+123", chars) );
chars = new char[] {'.'};
assertEquals("I aM.Fine", WordUtils.capitalize("i aM.fine", chars) );
assertEquals("I Am.fine", WordUtils.capitalize("i am.fine", null) );
}
public void testCapitalizeFully_String() {
assertEquals(null, WordUtils.capitalizeFully(null));
assertEquals("", WordUtils.capitalizeFully(""));
assertEquals(" ", WordUtils.capitalizeFully(" "));
assertEquals("I", WordUtils.capitalizeFully("I") );
assertEquals("I", WordUtils.capitalizeFully("i") );
assertEquals("I Am Here 123", WordUtils.capitalizeFully("i am here 123") );
assertEquals("I Am Here 123", WordUtils.capitalizeFully("I Am Here 123") );
assertEquals("I Am Here 123", WordUtils.capitalizeFully("i am HERE 123") );
assertEquals("I Am Here 123", WordUtils.capitalizeFully("I AM HERE 123") );
}
public void testCapitalizeFullyWithDelimiters_String() {
assertEquals(null, WordUtils.capitalizeFully(null, null));
assertEquals("", WordUtils.capitalizeFully("", new char[0]));
assertEquals(" ", WordUtils.capitalizeFully(" ", new char[0]));
char[] chars = new char[] { '-', '+', ' ', '@' };
assertEquals("I", WordUtils.capitalizeFully("I", chars) );
assertEquals("I", WordUtils.capitalizeFully("i", chars) );
assertEquals("I-Am Here+123", WordUtils.capitalizeFully("i-am here+123", chars) );
assertEquals("I Am+Here-123", WordUtils.capitalizeFully("I Am+Here-123", chars) );
assertEquals("I+Am-Here 123", WordUtils.capitalizeFully("i+am-HERE 123", chars) );
assertEquals("I-Am Here+123", WordUtils.capitalizeFully("I-AM HERE+123", chars) );
chars = new char[] {'.'};
assertEquals("I am.Fine", WordUtils.capitalizeFully("i aM.fine", chars) );
assertEquals("I Am.fine", WordUtils.capitalizeFully("i am.fine", null) );
}
public void testUncapitalize_String() {
assertEquals(null, WordUtils.uncapitalize(null));
assertEquals("", WordUtils.uncapitalize(""));
assertEquals(" ", WordUtils.uncapitalize(" "));
assertEquals("i", WordUtils.uncapitalize("I") );
assertEquals("i", WordUtils.uncapitalize("i") );
assertEquals("i am here 123", WordUtils.uncapitalize("i am here 123") );
assertEquals("i am here 123", WordUtils.uncapitalize("I Am Here 123") );
assertEquals("i am hERE 123", WordUtils.uncapitalize("i am HERE 123") );
assertEquals("i aM hERE 123", WordUtils.uncapitalize("I AM HERE 123") );
}
public void testUncapitalizeWithDelimiters_String() {
assertEquals(null, WordUtils.uncapitalize(null, null));
assertEquals("", WordUtils.uncapitalize("", new char[0]));
assertEquals(" ", WordUtils.uncapitalize(" ", new char[0]));
char[] chars = new char[] { '-', '+', ' ', '@' };
assertEquals("i", WordUtils.uncapitalize("I", chars) );
assertEquals("i", WordUtils.uncapitalize("i", chars) );
assertEquals("i am-here+123", WordUtils.uncapitalize("i am-here+123", chars) );
assertEquals("i+am here-123", WordUtils.uncapitalize("I+Am Here-123", chars) );
assertEquals("i-am+hERE 123", WordUtils.uncapitalize("i-am+HERE 123", chars) );
assertEquals("i aM-hERE+123", WordUtils.uncapitalize("I AM-HERE+123", chars) );
chars = new char[] {'.'};
assertEquals("i AM.fINE", WordUtils.uncapitalize("I AM.FINE", chars) );
assertEquals("i aM.FINE", WordUtils.uncapitalize("I AM.FINE", null) );
}
//-----------------------------------------------------------------------
public void testInitials_String() {
assertEquals(null, WordUtils.initials(null));
assertEquals("", WordUtils.initials(""));
assertEquals("", WordUtils.initials(" "));
assertEquals("I", WordUtils.initials("I"));
assertEquals("i", WordUtils.initials("i"));
assertEquals("BJL", WordUtils.initials("Ben John Lee"));
assertEquals("BJ", WordUtils.initials("Ben J.Lee"));
assertEquals("BJ.L", WordUtils.initials(" Ben John . Lee"));
assertEquals("iah1", WordUtils.initials("i am here 123"));
}
// -----------------------------------------------------------------------
public void testInitials_String_charArray() {
char[] array = null;
assertEquals(null, WordUtils.initials(null, array));
assertEquals("", WordUtils.initials("", array));
assertEquals("", WordUtils.initials(" ", array));
assertEquals("I", WordUtils.initials("I", array));
assertEquals("i", WordUtils.initials("i", array));
assertEquals("S", WordUtils.initials("SJC", array));
assertEquals("BJL", WordUtils.initials("Ben John Lee", array));
assertEquals("BJ", WordUtils.initials("Ben J.Lee", array));
assertEquals("BJ.L", WordUtils.initials(" Ben John . Lee", array));
assertEquals("KO", WordUtils.initials("Kay O'Murphy", array));
assertEquals("iah1", WordUtils.initials("i am here 123", array));
array = new char[0];
assertEquals(null, WordUtils.initials(null, array));
assertEquals("", WordUtils.initials("", array));
assertEquals("", WordUtils.initials(" ", array));
assertEquals("", WordUtils.initials("I", array));
assertEquals("", WordUtils.initials("i", array));
assertEquals("", WordUtils.initials("SJC", array));
assertEquals("", WordUtils.initials("Ben John Lee", array));
assertEquals("", WordUtils.initials("Ben J.Lee", array));
assertEquals("", WordUtils.initials(" Ben John . Lee", array));
assertEquals("", WordUtils.initials("Kay O'Murphy", array));
assertEquals("", WordUtils.initials("i am here 123", array));
array = " ".toCharArray();
assertEquals(null, WordUtils.initials(null, array));
assertEquals("", WordUtils.initials("", array));
assertEquals("", WordUtils.initials(" ", array));
assertEquals("I", WordUtils.initials("I", array));
assertEquals("i", WordUtils.initials("i", array));
assertEquals("S", WordUtils.initials("SJC", array));
assertEquals("BJL", WordUtils.initials("Ben John Lee", array));
assertEquals("BJ", WordUtils.initials("Ben J.Lee", array));
assertEquals("BJ.L", WordUtils.initials(" Ben John . Lee", array));
assertEquals("KO", WordUtils.initials("Kay O'Murphy", array));
assertEquals("iah1", WordUtils.initials("i am here 123", array));
array = " .".toCharArray();
assertEquals(null, WordUtils.initials(null, array));
assertEquals("", WordUtils.initials("", array));
assertEquals("", WordUtils.initials(" ", array));
assertEquals("I", WordUtils.initials("I", array));
assertEquals("i", WordUtils.initials("i", array));
assertEquals("S", WordUtils.initials("SJC", array));
assertEquals("BJL", WordUtils.initials("Ben John Lee", array));
assertEquals("BJL", WordUtils.initials("Ben J.Lee", array));
assertEquals("BJL", WordUtils.initials(" Ben John . Lee", array));
assertEquals("KO", WordUtils.initials("Kay O'Murphy", array));
assertEquals("iah1", WordUtils.initials("i am here 123", array));
array = " .'".toCharArray();
assertEquals(null, WordUtils.initials(null, array));
assertEquals("", WordUtils.initials("", array));
assertEquals("", WordUtils.initials(" ", array));
assertEquals("I", WordUtils.initials("I", array));
assertEquals("i", WordUtils.initials("i", array));
assertEquals("S", WordUtils.initials("SJC", array));
assertEquals("BJL", WordUtils.initials("Ben John Lee", array));
assertEquals("BJL", WordUtils.initials("Ben J.Lee", array));
assertEquals("BJL", WordUtils.initials(" Ben John . Lee", array));
assertEquals("KOM", WordUtils.initials("Kay O'Murphy", array));
assertEquals("iah1", WordUtils.initials("i am here 123", array));
array = "SIJo1".toCharArray();
assertEquals(null, WordUtils.initials(null, array));
assertEquals("", WordUtils.initials("", array));
assertEquals(" ", WordUtils.initials(" ", array));
assertEquals("", WordUtils.initials("I", array));
assertEquals("i", WordUtils.initials("i", array));
assertEquals("C", WordUtils.initials("SJC", array));
assertEquals("Bh", WordUtils.initials("Ben John Lee", array));
assertEquals("B.", WordUtils.initials("Ben J.Lee", array));
assertEquals(" h", WordUtils.initials(" Ben John . Lee", array));
assertEquals("K", WordUtils.initials("Kay O'Murphy", array));
assertEquals("i2", WordUtils.initials("i am here 123", array));
}
// -----------------------------------------------------------------------
public void testSwapCase_String() {
assertEquals(null, WordUtils.swapCase(null));
assertEquals("", WordUtils.swapCase(""));
assertEquals(" ", WordUtils.swapCase(" "));
assertEquals("i", WordUtils.swapCase("I") );
assertEquals("I", WordUtils.swapCase("i") );
assertEquals("I AM HERE 123", WordUtils.swapCase("i am here 123") );
assertEquals("i aM hERE 123", WordUtils.swapCase("I Am Here 123") );
assertEquals("I AM here 123", WordUtils.swapCase("i am HERE 123") );
assertEquals("i am here 123", WordUtils.swapCase("I AM HERE 123") );
String test = "This String contains a TitleCase character: \u01C8";
String expect = "tHIS sTRING CONTAINS A tITLEcASE CHARACTER: \u01C9";
assertEquals(expect, WordUtils.swapCase(test));
}
// -----------------------------------------------------------------------
public void testAbbreviate() {
// check null and empty are returned respectively
assertNull(WordUtils.abbreviate(null, 1,-1,""));
assertEquals(StringUtils.EMPTY, WordUtils.abbreviate("", 1,-1,""));
// test upper limit
assertEquals("01234", WordUtils.abbreviate("0123456789", 0,5,""));
assertEquals("01234", WordUtils.abbreviate("0123456789", 5, 2,""));
assertEquals("012", WordUtils.abbreviate("012 3456789", 2, 5,""));
assertEquals("012 3", WordUtils.abbreviate("012 3456789", 5, 2,""));
assertEquals("0123456789", WordUtils.abbreviate("0123456789", 0,-1,""));
// test upper limit + append string
assertEquals("01234-", WordUtils.abbreviate("0123456789", 0,5,"-"));
assertEquals("01234-", WordUtils.abbreviate("0123456789", 5, 2,"-"));
assertEquals("012", WordUtils.abbreviate("012 3456789", 2, 5, null));
assertEquals("012 3", WordUtils.abbreviate("012 3456789", 5, 2,""));
assertEquals("0123456789", WordUtils.abbreviate("0123456789", 0,-1,""));
// test lower value
assertEquals("012", WordUtils.abbreviate("012 3456789", 0,5, null));
assertEquals("01234", WordUtils.abbreviate("01234 56789", 5, 10, null));
assertEquals("01 23 45 67", WordUtils.abbreviate("01 23 45 67 89", 9, -1, null));
assertEquals("01 23 45 6", WordUtils.abbreviate("01 23 45 67 89", 9, 10, null));
assertEquals("0123456789", WordUtils.abbreviate("0123456789", 15, 20, null));
// test lower value + append
assertEquals("012", WordUtils.abbreviate("012 3456789", 0,5, null));
assertEquals("01234-", WordUtils.abbreviate("01234 56789", 5, 10, "-"));
assertEquals("01 23 45 67abc", WordUtils.abbreviate("01 23 45 67 89", 9, -1, "abc"));
assertEquals("01 23 45 6", WordUtils.abbreviate("01 23 45 67 89", 9, 10, ""));
// others
assertEquals("", WordUtils.abbreviate("0123456790", 0,0,""));
assertEquals("", WordUtils.abbreviate(" 0123456790", 0,-1,""));
}
} |
|
@Test
public void testSmallDistances() {
// Create a bunch of CloseIntegerPoints. Most are identical, but one is different by a
// small distance.
int[] repeatedArray = { 0 };
int[] uniqueArray = { 1 };
CloseIntegerPoint repeatedPoint =
new CloseIntegerPoint(new EuclideanIntegerPoint(repeatedArray));
CloseIntegerPoint uniquePoint =
new CloseIntegerPoint(new EuclideanIntegerPoint(uniqueArray));
Collection<CloseIntegerPoint> points = new ArrayList<CloseIntegerPoint>();
final int NUM_REPEATED_POINTS = 10 * 1000;
for (int i = 0; i < NUM_REPEATED_POINTS; ++i) {
points.add(repeatedPoint);
}
points.add(uniquePoint);
// Ask a KMeansPlusPlusClusterer to run zero iterations (i.e., to simply choose initial
// cluster centers).
final long RANDOM_SEED = 0;
final int NUM_CLUSTERS = 2;
final int NUM_ITERATIONS = 0;
KMeansPlusPlusClusterer<CloseIntegerPoint> clusterer =
new KMeansPlusPlusClusterer<CloseIntegerPoint>(new Random(RANDOM_SEED));
List<Cluster<CloseIntegerPoint>> clusters =
clusterer.cluster(points, NUM_CLUSTERS, NUM_ITERATIONS);
// Check that one of the chosen centers is the unique point.
boolean uniquePointIsCenter = false;
for (Cluster<CloseIntegerPoint> cluster : clusters) {
if (cluster.getCenter().equals(uniquePoint)) {
uniquePointIsCenter = true;
}
}
assertTrue(uniquePointIsCenter);
} | org.apache.commons.math.stat.clustering.KMeansPlusPlusClustererTest::testSmallDistances | src/test/java/org/apache/commons/math/stat/clustering/KMeansPlusPlusClustererTest.java | 249 | src/test/java/org/apache/commons/math/stat/clustering/KMeansPlusPlusClustererTest.java | testSmallDistances | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.math.stat.clustering;
import static org.junit.Assert.assertEquals;
import static org.junit.Assert.assertTrue;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Random;
import org.junit.Assert;
import org.junit.Test;
public class KMeansPlusPlusClustererTest {
@Test
public void dimension2() {
KMeansPlusPlusClusterer<EuclideanIntegerPoint> transformer =
new KMeansPlusPlusClusterer<EuclideanIntegerPoint>(new Random(1746432956321l));
EuclideanIntegerPoint[] points = new EuclideanIntegerPoint[] {
// first expected cluster
new EuclideanIntegerPoint(new int[] { -15, 3 }),
new EuclideanIntegerPoint(new int[] { -15, 4 }),
new EuclideanIntegerPoint(new int[] { -15, 5 }),
new EuclideanIntegerPoint(new int[] { -14, 3 }),
new EuclideanIntegerPoint(new int[] { -14, 5 }),
new EuclideanIntegerPoint(new int[] { -13, 3 }),
new EuclideanIntegerPoint(new int[] { -13, 4 }),
new EuclideanIntegerPoint(new int[] { -13, 5 }),
// second expected cluster
new EuclideanIntegerPoint(new int[] { -1, 0 }),
new EuclideanIntegerPoint(new int[] { -1, -1 }),
new EuclideanIntegerPoint(new int[] { 0, -1 }),
new EuclideanIntegerPoint(new int[] { 1, -1 }),
new EuclideanIntegerPoint(new int[] { 1, -2 }),
// third expected cluster
new EuclideanIntegerPoint(new int[] { 13, 3 }),
new EuclideanIntegerPoint(new int[] { 13, 4 }),
new EuclideanIntegerPoint(new int[] { 14, 4 }),
new EuclideanIntegerPoint(new int[] { 14, 7 }),
new EuclideanIntegerPoint(new int[] { 16, 5 }),
new EuclideanIntegerPoint(new int[] { 16, 6 }),
new EuclideanIntegerPoint(new int[] { 17, 4 }),
new EuclideanIntegerPoint(new int[] { 17, 7 })
};
List<Cluster<EuclideanIntegerPoint>> clusters =
transformer.cluster(Arrays.asList(points), 3, 10);
assertEquals(3, clusters.size());
boolean cluster1Found = false;
boolean cluster2Found = false;
boolean cluster3Found = false;
for (Cluster<EuclideanIntegerPoint> cluster : clusters) {
int[] center = cluster.getCenter().getPoint();
if (center[0] < 0) {
cluster1Found = true;
assertEquals(8, cluster.getPoints().size());
assertEquals(-14, center[0]);
assertEquals( 4, center[1]);
} else if (center[1] < 0) {
cluster2Found = true;
assertEquals(5, cluster.getPoints().size());
assertEquals( 0, center[0]);
assertEquals(-1, center[1]);
} else {
cluster3Found = true;
assertEquals(8, cluster.getPoints().size());
assertEquals(15, center[0]);
assertEquals(5, center[1]);
}
}
assertTrue(cluster1Found);
assertTrue(cluster2Found);
assertTrue(cluster3Found);
}
/**
* JIRA: MATH-305
*
* Two points, one cluster, one iteration
*/
@Test
public void testPerformClusterAnalysisDegenerate() {
KMeansPlusPlusClusterer<EuclideanIntegerPoint> transformer = new KMeansPlusPlusClusterer<EuclideanIntegerPoint>(
new Random(1746432956321l));
EuclideanIntegerPoint[] points = new EuclideanIntegerPoint[] {
new EuclideanIntegerPoint(new int[] { 1959, 325100 }),
new EuclideanIntegerPoint(new int[] { 1960, 373200 }), };
List<Cluster<EuclideanIntegerPoint>> clusters = transformer.cluster(Arrays.asList(points), 1, 1);
assertEquals(1, clusters.size());
assertEquals(2, (clusters.get(0).getPoints().size()));
EuclideanIntegerPoint pt1 = new EuclideanIntegerPoint(new int[] { 1959, 325100 });
EuclideanIntegerPoint pt2 = new EuclideanIntegerPoint(new int[] { 1960, 373200 });
assertTrue(clusters.get(0).getPoints().contains(pt1));
assertTrue(clusters.get(0).getPoints().contains(pt2));
}
@Test
public void testCertainSpace() {
KMeansPlusPlusClusterer.EmptyClusterStrategy[] strategies = {
KMeansPlusPlusClusterer.EmptyClusterStrategy.LARGEST_VARIANCE,
KMeansPlusPlusClusterer.EmptyClusterStrategy.LARGEST_POINTS_NUMBER,
KMeansPlusPlusClusterer.EmptyClusterStrategy.FARTHEST_POINT
};
for (KMeansPlusPlusClusterer.EmptyClusterStrategy strategy : strategies) {
KMeansPlusPlusClusterer<EuclideanIntegerPoint> transformer =
new KMeansPlusPlusClusterer<EuclideanIntegerPoint>(new Random(1746432956321l), strategy);
int numberOfVariables = 27;
// initialise testvalues
int position1 = 1;
int position2 = position1 + numberOfVariables;
int position3 = position2 + numberOfVariables;
int position4 = position3 + numberOfVariables;
// testvalues will be multiplied
int multiplier = 1000000;
EuclideanIntegerPoint[] breakingPoints = new EuclideanIntegerPoint[numberOfVariables];
// define the space which will break the cluster algorithm
for (int i = 0; i < numberOfVariables; i++) {
int points[] = { position1, position2, position3, position4 };
// multiply the values
for (int j = 0; j < points.length; j++) {
points[j] = points[j] * multiplier;
}
EuclideanIntegerPoint euclideanIntegerPoint = new EuclideanIntegerPoint(points);
breakingPoints[i] = euclideanIntegerPoint;
position1 = position1 + numberOfVariables;
position2 = position2 + numberOfVariables;
position3 = position3 + numberOfVariables;
position4 = position4 + numberOfVariables;
}
for (int n = 2; n < 27; ++n) {
List<Cluster<EuclideanIntegerPoint>> clusters =
transformer.cluster(Arrays.asList(breakingPoints), n, 100);
Assert.assertEquals(n, clusters.size());
int sum = 0;
for (Cluster<EuclideanIntegerPoint> cluster : clusters) {
sum += cluster.getPoints().size();
}
Assert.assertEquals(numberOfVariables, sum);
}
}
}
/**
* A helper class for testSmallDistances(). This class is similar to EuclideanIntegerPoint, but
* it defines a different distanceFrom() method that tends to return distances less than 1.
*/
private class CloseIntegerPoint implements Clusterable<CloseIntegerPoint> {
public CloseIntegerPoint(EuclideanIntegerPoint point) {
euclideanPoint = point;
}
public double distanceFrom(CloseIntegerPoint p) {
return euclideanPoint.distanceFrom(p.euclideanPoint) * 0.001;
}
public CloseIntegerPoint centroidOf(Collection<CloseIntegerPoint> p) {
Collection<EuclideanIntegerPoint> euclideanPoints =
new ArrayList<EuclideanIntegerPoint>();
for (CloseIntegerPoint point : p) {
euclideanPoints.add(point.euclideanPoint);
}
return new CloseIntegerPoint(euclideanPoint.centroidOf(euclideanPoints));
}
@Override
public boolean equals(Object o) {
if (!(o instanceof CloseIntegerPoint)) {
return false;
}
CloseIntegerPoint p = (CloseIntegerPoint) o;
return euclideanPoint.equals(p.euclideanPoint);
}
@Override
public int hashCode() {
return euclideanPoint.hashCode();
}
private EuclideanIntegerPoint euclideanPoint;
}
/**
* Test points that are very close together. See issue MATH-546.
*/
@Test
public void testSmallDistances() {
// Create a bunch of CloseIntegerPoints. Most are identical, but one is different by a
// small distance.
int[] repeatedArray = { 0 };
int[] uniqueArray = { 1 };
CloseIntegerPoint repeatedPoint =
new CloseIntegerPoint(new EuclideanIntegerPoint(repeatedArray));
CloseIntegerPoint uniquePoint =
new CloseIntegerPoint(new EuclideanIntegerPoint(uniqueArray));
Collection<CloseIntegerPoint> points = new ArrayList<CloseIntegerPoint>();
final int NUM_REPEATED_POINTS = 10 * 1000;
for (int i = 0; i < NUM_REPEATED_POINTS; ++i) {
points.add(repeatedPoint);
}
points.add(uniquePoint);
// Ask a KMeansPlusPlusClusterer to run zero iterations (i.e., to simply choose initial
// cluster centers).
final long RANDOM_SEED = 0;
final int NUM_CLUSTERS = 2;
final int NUM_ITERATIONS = 0;
KMeansPlusPlusClusterer<CloseIntegerPoint> clusterer =
new KMeansPlusPlusClusterer<CloseIntegerPoint>(new Random(RANDOM_SEED));
List<Cluster<CloseIntegerPoint>> clusters =
clusterer.cluster(points, NUM_CLUSTERS, NUM_ITERATIONS);
// Check that one of the chosen centers is the unique point.
boolean uniquePointIsCenter = false;
for (Cluster<CloseIntegerPoint> cluster : clusters) {
if (cluster.getCenter().equals(uniquePoint)) {
uniquePointIsCenter = true;
}
}
assertTrue(uniquePointIsCenter);
}
} | // You are a professional Java test case writer, please create a test case named `testSmallDistances` for the issue `Math-MATH-546`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Math-MATH-546
//
// ## Issue-Title:
// Truncation issue in KMeansPlusPlusClusterer
//
// ## Issue-Description:
//
// The for loop inside KMeansPlusPlusClusterer.chooseInitialClusters defines a variable
//
// int sum = 0;
//
// This variable should have type double, rather than int. Using an int causes the method to truncate the distances between points to (square roots of) integers. It's especially bad when the distances between points are typically less than 1.
//
//
// As an aside, in version 2.2, this bug manifested itself by making the clusterer return empty clusters. I wonder if the EmptyClusterStrategy would still be necessary if this bug were fixed.
//
//
//
//
//
@Test
public void testSmallDistances() {
| 249 | /**
* Test points that are very close together. See issue MATH-546.
*/ | 57 | 213 | src/test/java/org/apache/commons/math/stat/clustering/KMeansPlusPlusClustererTest.java | src/test/java | ```markdown
## Issue-ID: Math-MATH-546
## Issue-Title:
Truncation issue in KMeansPlusPlusClusterer
## Issue-Description:
The for loop inside KMeansPlusPlusClusterer.chooseInitialClusters defines a variable
int sum = 0;
This variable should have type double, rather than int. Using an int causes the method to truncate the distances between points to (square roots of) integers. It's especially bad when the distances between points are typically less than 1.
As an aside, in version 2.2, this bug manifested itself by making the clusterer return empty clusters. I wonder if the EmptyClusterStrategy would still be necessary if this bug were fixed.
```
You are a professional Java test case writer, please create a test case named `testSmallDistances` for the issue `Math-MATH-546`, utilizing the provided issue report information and the following function signature.
```java
@Test
public void testSmallDistances() {
```
| 213 | [
"org.apache.commons.math.stat.clustering.KMeansPlusPlusClusterer"
] | 71de60a0306b7aa5a2e1e3d9470587b4a8932733c9c2bad2d339f21da06966e2 | @Test
public void testSmallDistances() | // You are a professional Java test case writer, please create a test case named `testSmallDistances` for the issue `Math-MATH-546`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Math-MATH-546
//
// ## Issue-Title:
// Truncation issue in KMeansPlusPlusClusterer
//
// ## Issue-Description:
//
// The for loop inside KMeansPlusPlusClusterer.chooseInitialClusters defines a variable
//
// int sum = 0;
//
// This variable should have type double, rather than int. Using an int causes the method to truncate the distances between points to (square roots of) integers. It's especially bad when the distances between points are typically less than 1.
//
//
// As an aside, in version 2.2, this bug manifested itself by making the clusterer return empty clusters. I wonder if the EmptyClusterStrategy would still be necessary if this bug were fixed.
//
//
//
//
//
| Math | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.math.stat.clustering;
import static org.junit.Assert.assertEquals;
import static org.junit.Assert.assertTrue;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Random;
import org.junit.Assert;
import org.junit.Test;
public class KMeansPlusPlusClustererTest {
@Test
public void dimension2() {
KMeansPlusPlusClusterer<EuclideanIntegerPoint> transformer =
new KMeansPlusPlusClusterer<EuclideanIntegerPoint>(new Random(1746432956321l));
EuclideanIntegerPoint[] points = new EuclideanIntegerPoint[] {
// first expected cluster
new EuclideanIntegerPoint(new int[] { -15, 3 }),
new EuclideanIntegerPoint(new int[] { -15, 4 }),
new EuclideanIntegerPoint(new int[] { -15, 5 }),
new EuclideanIntegerPoint(new int[] { -14, 3 }),
new EuclideanIntegerPoint(new int[] { -14, 5 }),
new EuclideanIntegerPoint(new int[] { -13, 3 }),
new EuclideanIntegerPoint(new int[] { -13, 4 }),
new EuclideanIntegerPoint(new int[] { -13, 5 }),
// second expected cluster
new EuclideanIntegerPoint(new int[] { -1, 0 }),
new EuclideanIntegerPoint(new int[] { -1, -1 }),
new EuclideanIntegerPoint(new int[] { 0, -1 }),
new EuclideanIntegerPoint(new int[] { 1, -1 }),
new EuclideanIntegerPoint(new int[] { 1, -2 }),
// third expected cluster
new EuclideanIntegerPoint(new int[] { 13, 3 }),
new EuclideanIntegerPoint(new int[] { 13, 4 }),
new EuclideanIntegerPoint(new int[] { 14, 4 }),
new EuclideanIntegerPoint(new int[] { 14, 7 }),
new EuclideanIntegerPoint(new int[] { 16, 5 }),
new EuclideanIntegerPoint(new int[] { 16, 6 }),
new EuclideanIntegerPoint(new int[] { 17, 4 }),
new EuclideanIntegerPoint(new int[] { 17, 7 })
};
List<Cluster<EuclideanIntegerPoint>> clusters =
transformer.cluster(Arrays.asList(points), 3, 10);
assertEquals(3, clusters.size());
boolean cluster1Found = false;
boolean cluster2Found = false;
boolean cluster3Found = false;
for (Cluster<EuclideanIntegerPoint> cluster : clusters) {
int[] center = cluster.getCenter().getPoint();
if (center[0] < 0) {
cluster1Found = true;
assertEquals(8, cluster.getPoints().size());
assertEquals(-14, center[0]);
assertEquals( 4, center[1]);
} else if (center[1] < 0) {
cluster2Found = true;
assertEquals(5, cluster.getPoints().size());
assertEquals( 0, center[0]);
assertEquals(-1, center[1]);
} else {
cluster3Found = true;
assertEquals(8, cluster.getPoints().size());
assertEquals(15, center[0]);
assertEquals(5, center[1]);
}
}
assertTrue(cluster1Found);
assertTrue(cluster2Found);
assertTrue(cluster3Found);
}
/**
* JIRA: MATH-305
*
* Two points, one cluster, one iteration
*/
@Test
public void testPerformClusterAnalysisDegenerate() {
KMeansPlusPlusClusterer<EuclideanIntegerPoint> transformer = new KMeansPlusPlusClusterer<EuclideanIntegerPoint>(
new Random(1746432956321l));
EuclideanIntegerPoint[] points = new EuclideanIntegerPoint[] {
new EuclideanIntegerPoint(new int[] { 1959, 325100 }),
new EuclideanIntegerPoint(new int[] { 1960, 373200 }), };
List<Cluster<EuclideanIntegerPoint>> clusters = transformer.cluster(Arrays.asList(points), 1, 1);
assertEquals(1, clusters.size());
assertEquals(2, (clusters.get(0).getPoints().size()));
EuclideanIntegerPoint pt1 = new EuclideanIntegerPoint(new int[] { 1959, 325100 });
EuclideanIntegerPoint pt2 = new EuclideanIntegerPoint(new int[] { 1960, 373200 });
assertTrue(clusters.get(0).getPoints().contains(pt1));
assertTrue(clusters.get(0).getPoints().contains(pt2));
}
@Test
public void testCertainSpace() {
KMeansPlusPlusClusterer.EmptyClusterStrategy[] strategies = {
KMeansPlusPlusClusterer.EmptyClusterStrategy.LARGEST_VARIANCE,
KMeansPlusPlusClusterer.EmptyClusterStrategy.LARGEST_POINTS_NUMBER,
KMeansPlusPlusClusterer.EmptyClusterStrategy.FARTHEST_POINT
};
for (KMeansPlusPlusClusterer.EmptyClusterStrategy strategy : strategies) {
KMeansPlusPlusClusterer<EuclideanIntegerPoint> transformer =
new KMeansPlusPlusClusterer<EuclideanIntegerPoint>(new Random(1746432956321l), strategy);
int numberOfVariables = 27;
// initialise testvalues
int position1 = 1;
int position2 = position1 + numberOfVariables;
int position3 = position2 + numberOfVariables;
int position4 = position3 + numberOfVariables;
// testvalues will be multiplied
int multiplier = 1000000;
EuclideanIntegerPoint[] breakingPoints = new EuclideanIntegerPoint[numberOfVariables];
// define the space which will break the cluster algorithm
for (int i = 0; i < numberOfVariables; i++) {
int points[] = { position1, position2, position3, position4 };
// multiply the values
for (int j = 0; j < points.length; j++) {
points[j] = points[j] * multiplier;
}
EuclideanIntegerPoint euclideanIntegerPoint = new EuclideanIntegerPoint(points);
breakingPoints[i] = euclideanIntegerPoint;
position1 = position1 + numberOfVariables;
position2 = position2 + numberOfVariables;
position3 = position3 + numberOfVariables;
position4 = position4 + numberOfVariables;
}
for (int n = 2; n < 27; ++n) {
List<Cluster<EuclideanIntegerPoint>> clusters =
transformer.cluster(Arrays.asList(breakingPoints), n, 100);
Assert.assertEquals(n, clusters.size());
int sum = 0;
for (Cluster<EuclideanIntegerPoint> cluster : clusters) {
sum += cluster.getPoints().size();
}
Assert.assertEquals(numberOfVariables, sum);
}
}
}
/**
* A helper class for testSmallDistances(). This class is similar to EuclideanIntegerPoint, but
* it defines a different distanceFrom() method that tends to return distances less than 1.
*/
private class CloseIntegerPoint implements Clusterable<CloseIntegerPoint> {
public CloseIntegerPoint(EuclideanIntegerPoint point) {
euclideanPoint = point;
}
public double distanceFrom(CloseIntegerPoint p) {
return euclideanPoint.distanceFrom(p.euclideanPoint) * 0.001;
}
public CloseIntegerPoint centroidOf(Collection<CloseIntegerPoint> p) {
Collection<EuclideanIntegerPoint> euclideanPoints =
new ArrayList<EuclideanIntegerPoint>();
for (CloseIntegerPoint point : p) {
euclideanPoints.add(point.euclideanPoint);
}
return new CloseIntegerPoint(euclideanPoint.centroidOf(euclideanPoints));
}
@Override
public boolean equals(Object o) {
if (!(o instanceof CloseIntegerPoint)) {
return false;
}
CloseIntegerPoint p = (CloseIntegerPoint) o;
return euclideanPoint.equals(p.euclideanPoint);
}
@Override
public int hashCode() {
return euclideanPoint.hashCode();
}
private EuclideanIntegerPoint euclideanPoint;
}
/**
* Test points that are very close together. See issue MATH-546.
*/
@Test
public void testSmallDistances() {
// Create a bunch of CloseIntegerPoints. Most are identical, but one is different by a
// small distance.
int[] repeatedArray = { 0 };
int[] uniqueArray = { 1 };
CloseIntegerPoint repeatedPoint =
new CloseIntegerPoint(new EuclideanIntegerPoint(repeatedArray));
CloseIntegerPoint uniquePoint =
new CloseIntegerPoint(new EuclideanIntegerPoint(uniqueArray));
Collection<CloseIntegerPoint> points = new ArrayList<CloseIntegerPoint>();
final int NUM_REPEATED_POINTS = 10 * 1000;
for (int i = 0; i < NUM_REPEATED_POINTS; ++i) {
points.add(repeatedPoint);
}
points.add(uniquePoint);
// Ask a KMeansPlusPlusClusterer to run zero iterations (i.e., to simply choose initial
// cluster centers).
final long RANDOM_SEED = 0;
final int NUM_CLUSTERS = 2;
final int NUM_ITERATIONS = 0;
KMeansPlusPlusClusterer<CloseIntegerPoint> clusterer =
new KMeansPlusPlusClusterer<CloseIntegerPoint>(new Random(RANDOM_SEED));
List<Cluster<CloseIntegerPoint>> clusters =
clusterer.cluster(points, NUM_CLUSTERS, NUM_ITERATIONS);
// Check that one of the chosen centers is the unique point.
boolean uniquePointIsCenter = false;
for (Cluster<CloseIntegerPoint> cluster : clusters) {
if (cluster.getCenter().equals(uniquePoint)) {
uniquePointIsCenter = true;
}
}
assertTrue(uniquePointIsCenter);
}
} |
|
public void testIssue538() {
checkCompilesToSame( "/** @constructor */\n" +
"WebInspector.Setting = function() {}\n" +
"WebInspector.Setting.prototype = {\n" +
" get name0(){return this._name;},\n" +
" get name1(){return this._name;},\n" +
" get name2(){return this._name;},\n" +
" get name3(){return this._name;},\n" +
" get name4(){return this._name;},\n" +
" get name5(){return this._name;},\n" +
" get name6(){return this._name;},\n" +
" get name7(){return this._name;},\n" +
" get name8(){return this._name;},\n" +
" get name9(){return this._name;},\n" +
"}", 1);
} | com.google.javascript.jscomp.FunctionRewriterTest::testIssue538 | test/com/google/javascript/jscomp/FunctionRewriterTest.java | 179 | test/com/google/javascript/jscomp/FunctionRewriterTest.java | testIssue538 | /*
* Copyright 2009 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
/**
* Tests for {@link FunctionRewriter}
*
*/
public class FunctionRewriterTest extends CompilerTestCase {
private static final String RETURNARG_HELPER =
"function JSCompiler_returnArg(JSCompiler_returnArg_value){" +
" return function() { return JSCompiler_returnArg_value }" +
"}";
private static final String GET_HELPER =
"function JSCompiler_get(JSCompiler_get_name){" +
" return function() { return this[JSCompiler_get_name] }" +
"}";
private static final String SET_HELPER =
"function JSCompiler_set(JSCompiler_set_name) {" +
" return function(JSCompiler_set_value){" +
" this[JSCompiler_set_name]=JSCompiler_set_value" +
" }" +
"}";
private static final String EMPTY_HELPER =
"function JSCompiler_emptyFn() {" +
" return function(){}" +
"}";
private static final String IDENTITY_HELPER =
"function JSCompiler_identityFn() {" +
" return function(JSCompiler_identityFn_value) {" +
" return JSCompiler_identityFn_value" +
" }" +
"}";
@Override
protected void setUp() {
super.enableLineNumberCheck(false);
}
@Override
protected FunctionRewriter getProcessor(Compiler compiler) {
return new FunctionRewriter(compiler);
}
@Override
protected int getNumRepetitions() {
// Pass reaches steady state after just 1 iteration
return 1;
}
public void testReplaceReturnConst1() {
String source = "a.prototype.foo = function() {return \"foobar\"}";
checkCompilesToSame(source, 3);
checkCompilesTo(source,
RETURNARG_HELPER,
"a.prototype.foo = JSCompiler_returnArg(\"foobar\")",
4);
}
public void testReplaceReturnConst2() {
checkCompilesToSame("a.prototype.foo = function() {return foobar}", 10);
}
public void testReplaceReturnConst3() {
String source = "a.prototype.foo = function() {return void 0;}";
checkCompilesToSame(source, 3);
checkCompilesTo(source,
RETURNARG_HELPER,
"a.prototype.foo = JSCompiler_returnArg(void 0)",
4);
}
public void testReplaceGetter1() {
String source = "a.prototype.foo = function() {return this.foo_}";
checkCompilesToSame(source, 3);
checkCompilesTo(source,
GET_HELPER,
"a.prototype.foo = JSCompiler_get(\"foo_\")",
4);
}
public void testReplaceGetter2() {
checkCompilesToSame("a.prototype.foo = function() {return}", 10);
}
public void testReplaceSetter1() {
String source = "a.prototype.foo = function(v) {this.foo_ = v}";
checkCompilesToSame(source, 4);
checkCompilesTo(source,
SET_HELPER,
"a.prototype.foo = JSCompiler_set(\"foo_\")",
5);
}
public void testReplaceSetter2() {
String source = "a.prototype.foo = function(v, v2) {this.foo_ = v}";
checkCompilesToSame(source, 3);
checkCompilesTo(source,
SET_HELPER,
"a.prototype.foo = JSCompiler_set(\"foo_\")",
4);
}
public void testReplaceSetter3() {
checkCompilesToSame("a.prototype.foo = function() {this.foo_ = v}", 10);
}
public void testReplaceSetter4() {
checkCompilesToSame(
"a.prototype.foo = function(v, v2) {this.foo_ = v2}", 10);
}
public void testReplaceEmptyFunction1() {
String source = "a.prototype.foo = function() {}";
checkCompilesToSame(source, 4);
checkCompilesTo(source,
EMPTY_HELPER,
"a.prototype.foo = JSCompiler_emptyFn()",
5);
}
public void testReplaceEmptyFunction2() {
checkCompilesToSame("function foo() {}", 10);
}
public void testReplaceEmptyFunction3() {
String source = "var foo = function() {}";
checkCompilesToSame(source, 4);
checkCompilesTo(source,
EMPTY_HELPER,
"var foo = JSCompiler_emptyFn()",
5);
}
public void testReplaceIdentityFunction1() {
String source = "a.prototype.foo = function(a) {return a}";
checkCompilesToSame(source, 2);
checkCompilesTo(source,
IDENTITY_HELPER,
"a.prototype.foo = JSCompiler_identityFn()",
3);
}
public void testReplaceIdentityFunction2() {
checkCompilesToSame("a.prototype.foo = function(a) {return a + 1}", 10);
}
public void testIssue538() {
checkCompilesToSame( "/** @constructor */\n" +
"WebInspector.Setting = function() {}\n" +
"WebInspector.Setting.prototype = {\n" +
" get name0(){return this._name;},\n" +
" get name1(){return this._name;},\n" +
" get name2(){return this._name;},\n" +
" get name3(){return this._name;},\n" +
" get name4(){return this._name;},\n" +
" get name5(){return this._name;},\n" +
" get name6(){return this._name;},\n" +
" get name7(){return this._name;},\n" +
" get name8(){return this._name;},\n" +
" get name9(){return this._name;},\n" +
"}", 1);
}
private void checkCompilesTo(String src,
String expectedHdr,
String expectedBody,
int repetitions) {
StringBuilder srcBuffer = new StringBuilder();
StringBuilder expectedBuffer = new StringBuilder();
expectedBuffer.append(expectedHdr);
for (int idx = 0; idx < repetitions; idx++) {
if (idx != 0) {
srcBuffer.append(";");
expectedBuffer.append(";");
}
srcBuffer.append(src);
expectedBuffer.append(expectedBody);
}
test(srcBuffer.toString(), expectedBuffer.toString());
}
private void checkCompilesToSame(String src, int repetitions) {
checkCompilesTo(src, "", src, repetitions);
}
} | // You are a professional Java test case writer, please create a test case named `testIssue538` for the issue `Closure-538`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-538
//
// ## Issue-Title:
// Exception when emitting code containing getters
//
// ## Issue-Description:
// Consider the following source code: http://trac.webkit.org/browser/trunk/Source/WebCore/inspector/front-end/Settings.js#L123
//
// Following exception fires unless I remove the "get name()" getter from the code.
//
// java.lang.RuntimeException: java.lang.IllegalStateException: Expected function but was call Reference node CALL 128 [free\_call: 1] [source\_file: Settings.js]
// at com.google.javascript.jscomp.Compiler.runCallable(Compiler.java:629)
// at com.google.javascript.jscomp.Compiler.runInCompilerThread(Compiler.java:574)
// at com.google.javascript.jscomp.Compiler.compile(Compiler.java:556)
// at com.google.javascript.jscomp.Compiler.compile(Compiler.java:515)
// at com.google.javascript.jscomp.AbstractCommandLineRunner.doRun(AbstractCommandLineRunner.java:662)
// at com.google.javascript.jscomp.AbstractCommandLineRunner.run(AbstractCommandLineRunner.java:295)
// at com.google.javascript.jscomp.CommandLineRunner.main(CommandLineRunner.java:758)
// Caused by: java.lang.IllegalStateException: Expected function but was call Reference node CALL 128 [free\_call: 1] [source\_file: Settings.js]
// at com.google.javascript.jscomp.AstValidator$1.handleViolation(AstValidator.java:51)
// at com.google.javascript.jscomp.AstValidator.violation(AstValidator.java:763)
// at com.google.javascript.jscomp.AstValidator.validateNodeType(AstValidator.java:768)
// at com.google.javascript.jscomp.AstValidator.validateFunctionExpression(AstValidator.java:359)
// at com.google.javascript.jscomp.AstValidator.validateObjectLitGetKey(AstValidator.java:696)
// at com.google.javascript.jscomp.AstValidator.validateObjectLitKey(AstValidator.java:677)
// at com.google.javascript.jscomp.AstValidator.validateObjectLit(AstValidator.java:670)
// at com.google.javascript.jscomp.AstValidator.validateExpression(AstValidator.java:252)
// at com.google.javascript.jscomp.AstValidator.validateAssignmentExpression(AstValidator.java:603)
// at com.google.javascript.jscomp.AstValidator.validateExpression(AstValidator.java:219)
// at com.google.javascript.jscomp.AstValidator.validateExprStmt(AstValidator.java:476)
// at com.google.javascript.jscomp.AstValidator.validateStatement(AstValidator.java:126)
// at com.google.javascript.jscomp.AstValidator.validateScript(AstValidator.java:89)
// at com.google.javascript.jscomp.AstValidator.validateCodeRoot(AstValidator.java:79)
// at com.google.javascript.jscomp.AstValidator.process(AstValidator.java:63)
// at com.google.javascript.jscomp.PhaseOptimizer$PassFactoryDelegate.processInternal(PhaseOptimizer.java:273)
// at com.google.javascript.jscomp.PhaseOptimizer$NamedPass.process(PhaseOptimizer.java:250)
// at com.google.javascript.jscomp.PhaseOptimizer.process(PhaseOptimizer.java:168)
// at com.google.javascript.jscomp.Compiler.optimize(Compiler.java:1634)
// at com.google.javascript.jscomp.Compiler.compileInternal(Compiler.java:664)
// at com.google.javascript.jscomp.Compiler.access$000(Compiler.java:70)
// at com.google.javascript.jscomp.Compiler$1.call(Compiler.java:559)
// at com.google.javascript.jscomp.
public void testIssue538() {
| 179 | 55 | 164 | test/com/google/javascript/jscomp/FunctionRewriterTest.java | test | ```markdown
## Issue-ID: Closure-538
## Issue-Title:
Exception when emitting code containing getters
## Issue-Description:
Consider the following source code: http://trac.webkit.org/browser/trunk/Source/WebCore/inspector/front-end/Settings.js#L123
Following exception fires unless I remove the "get name()" getter from the code.
java.lang.RuntimeException: java.lang.IllegalStateException: Expected function but was call Reference node CALL 128 [free\_call: 1] [source\_file: Settings.js]
at com.google.javascript.jscomp.Compiler.runCallable(Compiler.java:629)
at com.google.javascript.jscomp.Compiler.runInCompilerThread(Compiler.java:574)
at com.google.javascript.jscomp.Compiler.compile(Compiler.java:556)
at com.google.javascript.jscomp.Compiler.compile(Compiler.java:515)
at com.google.javascript.jscomp.AbstractCommandLineRunner.doRun(AbstractCommandLineRunner.java:662)
at com.google.javascript.jscomp.AbstractCommandLineRunner.run(AbstractCommandLineRunner.java:295)
at com.google.javascript.jscomp.CommandLineRunner.main(CommandLineRunner.java:758)
Caused by: java.lang.IllegalStateException: Expected function but was call Reference node CALL 128 [free\_call: 1] [source\_file: Settings.js]
at com.google.javascript.jscomp.AstValidator$1.handleViolation(AstValidator.java:51)
at com.google.javascript.jscomp.AstValidator.violation(AstValidator.java:763)
at com.google.javascript.jscomp.AstValidator.validateNodeType(AstValidator.java:768)
at com.google.javascript.jscomp.AstValidator.validateFunctionExpression(AstValidator.java:359)
at com.google.javascript.jscomp.AstValidator.validateObjectLitGetKey(AstValidator.java:696)
at com.google.javascript.jscomp.AstValidator.validateObjectLitKey(AstValidator.java:677)
at com.google.javascript.jscomp.AstValidator.validateObjectLit(AstValidator.java:670)
at com.google.javascript.jscomp.AstValidator.validateExpression(AstValidator.java:252)
at com.google.javascript.jscomp.AstValidator.validateAssignmentExpression(AstValidator.java:603)
at com.google.javascript.jscomp.AstValidator.validateExpression(AstValidator.java:219)
at com.google.javascript.jscomp.AstValidator.validateExprStmt(AstValidator.java:476)
at com.google.javascript.jscomp.AstValidator.validateStatement(AstValidator.java:126)
at com.google.javascript.jscomp.AstValidator.validateScript(AstValidator.java:89)
at com.google.javascript.jscomp.AstValidator.validateCodeRoot(AstValidator.java:79)
at com.google.javascript.jscomp.AstValidator.process(AstValidator.java:63)
at com.google.javascript.jscomp.PhaseOptimizer$PassFactoryDelegate.processInternal(PhaseOptimizer.java:273)
at com.google.javascript.jscomp.PhaseOptimizer$NamedPass.process(PhaseOptimizer.java:250)
at com.google.javascript.jscomp.PhaseOptimizer.process(PhaseOptimizer.java:168)
at com.google.javascript.jscomp.Compiler.optimize(Compiler.java:1634)
at com.google.javascript.jscomp.Compiler.compileInternal(Compiler.java:664)
at com.google.javascript.jscomp.Compiler.access$000(Compiler.java:70)
at com.google.javascript.jscomp.Compiler$1.call(Compiler.java:559)
at com.google.javascript.jscomp.
```
You are a professional Java test case writer, please create a test case named `testIssue538` for the issue `Closure-538`, utilizing the provided issue report information and the following function signature.
```java
public void testIssue538() {
```
| 164 | [
"com.google.javascript.jscomp.FunctionRewriter"
] | 71e22c9d3d5d76d5b005bae8930233044989bdc5da425fb12e927d110a0400c0 | public void testIssue538() | // You are a professional Java test case writer, please create a test case named `testIssue538` for the issue `Closure-538`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-538
//
// ## Issue-Title:
// Exception when emitting code containing getters
//
// ## Issue-Description:
// Consider the following source code: http://trac.webkit.org/browser/trunk/Source/WebCore/inspector/front-end/Settings.js#L123
//
// Following exception fires unless I remove the "get name()" getter from the code.
//
// java.lang.RuntimeException: java.lang.IllegalStateException: Expected function but was call Reference node CALL 128 [free\_call: 1] [source\_file: Settings.js]
// at com.google.javascript.jscomp.Compiler.runCallable(Compiler.java:629)
// at com.google.javascript.jscomp.Compiler.runInCompilerThread(Compiler.java:574)
// at com.google.javascript.jscomp.Compiler.compile(Compiler.java:556)
// at com.google.javascript.jscomp.Compiler.compile(Compiler.java:515)
// at com.google.javascript.jscomp.AbstractCommandLineRunner.doRun(AbstractCommandLineRunner.java:662)
// at com.google.javascript.jscomp.AbstractCommandLineRunner.run(AbstractCommandLineRunner.java:295)
// at com.google.javascript.jscomp.CommandLineRunner.main(CommandLineRunner.java:758)
// Caused by: java.lang.IllegalStateException: Expected function but was call Reference node CALL 128 [free\_call: 1] [source\_file: Settings.js]
// at com.google.javascript.jscomp.AstValidator$1.handleViolation(AstValidator.java:51)
// at com.google.javascript.jscomp.AstValidator.violation(AstValidator.java:763)
// at com.google.javascript.jscomp.AstValidator.validateNodeType(AstValidator.java:768)
// at com.google.javascript.jscomp.AstValidator.validateFunctionExpression(AstValidator.java:359)
// at com.google.javascript.jscomp.AstValidator.validateObjectLitGetKey(AstValidator.java:696)
// at com.google.javascript.jscomp.AstValidator.validateObjectLitKey(AstValidator.java:677)
// at com.google.javascript.jscomp.AstValidator.validateObjectLit(AstValidator.java:670)
// at com.google.javascript.jscomp.AstValidator.validateExpression(AstValidator.java:252)
// at com.google.javascript.jscomp.AstValidator.validateAssignmentExpression(AstValidator.java:603)
// at com.google.javascript.jscomp.AstValidator.validateExpression(AstValidator.java:219)
// at com.google.javascript.jscomp.AstValidator.validateExprStmt(AstValidator.java:476)
// at com.google.javascript.jscomp.AstValidator.validateStatement(AstValidator.java:126)
// at com.google.javascript.jscomp.AstValidator.validateScript(AstValidator.java:89)
// at com.google.javascript.jscomp.AstValidator.validateCodeRoot(AstValidator.java:79)
// at com.google.javascript.jscomp.AstValidator.process(AstValidator.java:63)
// at com.google.javascript.jscomp.PhaseOptimizer$PassFactoryDelegate.processInternal(PhaseOptimizer.java:273)
// at com.google.javascript.jscomp.PhaseOptimizer$NamedPass.process(PhaseOptimizer.java:250)
// at com.google.javascript.jscomp.PhaseOptimizer.process(PhaseOptimizer.java:168)
// at com.google.javascript.jscomp.Compiler.optimize(Compiler.java:1634)
// at com.google.javascript.jscomp.Compiler.compileInternal(Compiler.java:664)
// at com.google.javascript.jscomp.Compiler.access$000(Compiler.java:70)
// at com.google.javascript.jscomp.Compiler$1.call(Compiler.java:559)
// at com.google.javascript.jscomp.
| Closure | /*
* Copyright 2009 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
/**
* Tests for {@link FunctionRewriter}
*
*/
public class FunctionRewriterTest extends CompilerTestCase {
private static final String RETURNARG_HELPER =
"function JSCompiler_returnArg(JSCompiler_returnArg_value){" +
" return function() { return JSCompiler_returnArg_value }" +
"}";
private static final String GET_HELPER =
"function JSCompiler_get(JSCompiler_get_name){" +
" return function() { return this[JSCompiler_get_name] }" +
"}";
private static final String SET_HELPER =
"function JSCompiler_set(JSCompiler_set_name) {" +
" return function(JSCompiler_set_value){" +
" this[JSCompiler_set_name]=JSCompiler_set_value" +
" }" +
"}";
private static final String EMPTY_HELPER =
"function JSCompiler_emptyFn() {" +
" return function(){}" +
"}";
private static final String IDENTITY_HELPER =
"function JSCompiler_identityFn() {" +
" return function(JSCompiler_identityFn_value) {" +
" return JSCompiler_identityFn_value" +
" }" +
"}";
@Override
protected void setUp() {
super.enableLineNumberCheck(false);
}
@Override
protected FunctionRewriter getProcessor(Compiler compiler) {
return new FunctionRewriter(compiler);
}
@Override
protected int getNumRepetitions() {
// Pass reaches steady state after just 1 iteration
return 1;
}
public void testReplaceReturnConst1() {
String source = "a.prototype.foo = function() {return \"foobar\"}";
checkCompilesToSame(source, 3);
checkCompilesTo(source,
RETURNARG_HELPER,
"a.prototype.foo = JSCompiler_returnArg(\"foobar\")",
4);
}
public void testReplaceReturnConst2() {
checkCompilesToSame("a.prototype.foo = function() {return foobar}", 10);
}
public void testReplaceReturnConst3() {
String source = "a.prototype.foo = function() {return void 0;}";
checkCompilesToSame(source, 3);
checkCompilesTo(source,
RETURNARG_HELPER,
"a.prototype.foo = JSCompiler_returnArg(void 0)",
4);
}
public void testReplaceGetter1() {
String source = "a.prototype.foo = function() {return this.foo_}";
checkCompilesToSame(source, 3);
checkCompilesTo(source,
GET_HELPER,
"a.prototype.foo = JSCompiler_get(\"foo_\")",
4);
}
public void testReplaceGetter2() {
checkCompilesToSame("a.prototype.foo = function() {return}", 10);
}
public void testReplaceSetter1() {
String source = "a.prototype.foo = function(v) {this.foo_ = v}";
checkCompilesToSame(source, 4);
checkCompilesTo(source,
SET_HELPER,
"a.prototype.foo = JSCompiler_set(\"foo_\")",
5);
}
public void testReplaceSetter2() {
String source = "a.prototype.foo = function(v, v2) {this.foo_ = v}";
checkCompilesToSame(source, 3);
checkCompilesTo(source,
SET_HELPER,
"a.prototype.foo = JSCompiler_set(\"foo_\")",
4);
}
public void testReplaceSetter3() {
checkCompilesToSame("a.prototype.foo = function() {this.foo_ = v}", 10);
}
public void testReplaceSetter4() {
checkCompilesToSame(
"a.prototype.foo = function(v, v2) {this.foo_ = v2}", 10);
}
public void testReplaceEmptyFunction1() {
String source = "a.prototype.foo = function() {}";
checkCompilesToSame(source, 4);
checkCompilesTo(source,
EMPTY_HELPER,
"a.prototype.foo = JSCompiler_emptyFn()",
5);
}
public void testReplaceEmptyFunction2() {
checkCompilesToSame("function foo() {}", 10);
}
public void testReplaceEmptyFunction3() {
String source = "var foo = function() {}";
checkCompilesToSame(source, 4);
checkCompilesTo(source,
EMPTY_HELPER,
"var foo = JSCompiler_emptyFn()",
5);
}
public void testReplaceIdentityFunction1() {
String source = "a.prototype.foo = function(a) {return a}";
checkCompilesToSame(source, 2);
checkCompilesTo(source,
IDENTITY_HELPER,
"a.prototype.foo = JSCompiler_identityFn()",
3);
}
public void testReplaceIdentityFunction2() {
checkCompilesToSame("a.prototype.foo = function(a) {return a + 1}", 10);
}
public void testIssue538() {
checkCompilesToSame( "/** @constructor */\n" +
"WebInspector.Setting = function() {}\n" +
"WebInspector.Setting.prototype = {\n" +
" get name0(){return this._name;},\n" +
" get name1(){return this._name;},\n" +
" get name2(){return this._name;},\n" +
" get name3(){return this._name;},\n" +
" get name4(){return this._name;},\n" +
" get name5(){return this._name;},\n" +
" get name6(){return this._name;},\n" +
" get name7(){return this._name;},\n" +
" get name8(){return this._name;},\n" +
" get name9(){return this._name;},\n" +
"}", 1);
}
private void checkCompilesTo(String src,
String expectedHdr,
String expectedBody,
int repetitions) {
StringBuilder srcBuffer = new StringBuilder();
StringBuilder expectedBuffer = new StringBuilder();
expectedBuffer.append(expectedHdr);
for (int idx = 0; idx < repetitions; idx++) {
if (idx != 0) {
srcBuffer.append(";");
expectedBuffer.append(";");
}
srcBuffer.append(src);
expectedBuffer.append(expectedBody);
}
test(srcBuffer.toString(), expectedBuffer.toString());
}
private void checkCompilesToSame(String src, int repetitions) {
checkCompilesTo(src, "", src, repetitions);
}
} |
||
@Test public void settersOnOrphanAttribute() {
Attribute attr = new Attribute("one", "two");
attr.setKey("three");
String oldVal = attr.setValue("four");
assertEquals("two", oldVal);
assertEquals("three", attr.getKey());
assertEquals("four", attr.getValue());
assertEquals(null, attr.parent);
} | org.jsoup.nodes.AttributeTest::settersOnOrphanAttribute | src/test/java/org/jsoup/nodes/AttributeTest.java | 48 | src/test/java/org/jsoup/nodes/AttributeTest.java | settersOnOrphanAttribute | package org.jsoup.nodes;
import org.jsoup.Jsoup;
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class AttributeTest {
@Test public void html() {
Attribute attr = new Attribute("key", "value &");
assertEquals("key=\"value &\"", attr.html());
assertEquals(attr.html(), attr.toString());
}
@Test public void testWithSupplementaryCharacterInAttributeKeyAndValue() {
String s = new String(Character.toChars(135361));
Attribute attr = new Attribute(s, "A" + s + "B");
assertEquals(s + "=\"A" + s + "B\"", attr.html());
assertEquals(attr.html(), attr.toString());
}
@Test(expected = IllegalArgumentException.class) public void validatesKeysNotEmpty() {
Attribute attr = new Attribute(" ", "Check");
}
@Test(expected = IllegalArgumentException.class) public void validatesKeysNotEmptyViaSet() {
Attribute attr = new Attribute("One", "Check");
attr.setKey(" ");
}
@Test public void booleanAttributesAreEmptyStringValues() {
Document doc = Jsoup.parse("<div hidden>");
Attributes attributes = doc.body().child(0).attributes();
assertEquals("", attributes.get("hidden"));
Attribute first = attributes.iterator().next();
assertEquals("hidden", first.getKey());
assertEquals("", first.getValue());
}
@Test public void settersOnOrphanAttribute() {
Attribute attr = new Attribute("one", "two");
attr.setKey("three");
String oldVal = attr.setValue("four");
assertEquals("two", oldVal);
assertEquals("three", attr.getKey());
assertEquals("four", attr.getValue());
assertEquals(null, attr.parent);
}
} | // You are a professional Java test case writer, please create a test case named `settersOnOrphanAttribute` for the issue `Jsoup-1107`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Jsoup-1107
//
// ## Issue-Title:
// NPE in Attribute.setValue() for attribute without parent
//
// ## Issue-Description:
//
// ```
// public String setValue(String val) {
// String oldVal = parent.get(this.key);
// if (parent != null) {
// int i = parent.indexOfKey(this.key);
// if (i != Attributes.NotFound)
// parent.vals[i] = val;
// }
// this.val = val;
// return oldVal;
// }
//
// ```
//
// Its useless to check `parent` for `null` after it has been dereferenced. I guess this is a copy-paste-bug:
//
//
//
// ```
// public void setKey(String key) {
// Validate.notNull(key);
// key = key.trim();
// Validate.notEmpty(key); // trimming could potentially make empty, so validate here
// if (parent != null) {
// int i = parent.indexOfKey(this.key);
// if (i != Attributes.NotFound)
// parent.keys[i] = key;
// }
// this.key = key;
// }
//
// ```
//
//
//
@Test public void settersOnOrphanAttribute() {
| 48 | 89 | 40 | src/test/java/org/jsoup/nodes/AttributeTest.java | src/test/java | ```markdown
## Issue-ID: Jsoup-1107
## Issue-Title:
NPE in Attribute.setValue() for attribute without parent
## Issue-Description:
```
public String setValue(String val) {
String oldVal = parent.get(this.key);
if (parent != null) {
int i = parent.indexOfKey(this.key);
if (i != Attributes.NotFound)
parent.vals[i] = val;
}
this.val = val;
return oldVal;
}
```
Its useless to check `parent` for `null` after it has been dereferenced. I guess this is a copy-paste-bug:
```
public void setKey(String key) {
Validate.notNull(key);
key = key.trim();
Validate.notEmpty(key); // trimming could potentially make empty, so validate here
if (parent != null) {
int i = parent.indexOfKey(this.key);
if (i != Attributes.NotFound)
parent.keys[i] = key;
}
this.key = key;
}
```
```
You are a professional Java test case writer, please create a test case named `settersOnOrphanAttribute` for the issue `Jsoup-1107`, utilizing the provided issue report information and the following function signature.
```java
@Test public void settersOnOrphanAttribute() {
```
| 40 | [
"org.jsoup.nodes.Attribute"
] | 7218ba878311628d74a6ff44cd4b2853662342fba3ed6a4d3804a2790323f24f | @Test public void settersOnOrphanAttribute() | // You are a professional Java test case writer, please create a test case named `settersOnOrphanAttribute` for the issue `Jsoup-1107`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Jsoup-1107
//
// ## Issue-Title:
// NPE in Attribute.setValue() for attribute without parent
//
// ## Issue-Description:
//
// ```
// public String setValue(String val) {
// String oldVal = parent.get(this.key);
// if (parent != null) {
// int i = parent.indexOfKey(this.key);
// if (i != Attributes.NotFound)
// parent.vals[i] = val;
// }
// this.val = val;
// return oldVal;
// }
//
// ```
//
// Its useless to check `parent` for `null` after it has been dereferenced. I guess this is a copy-paste-bug:
//
//
//
// ```
// public void setKey(String key) {
// Validate.notNull(key);
// key = key.trim();
// Validate.notEmpty(key); // trimming could potentially make empty, so validate here
// if (parent != null) {
// int i = parent.indexOfKey(this.key);
// if (i != Attributes.NotFound)
// parent.keys[i] = key;
// }
// this.key = key;
// }
//
// ```
//
//
//
| Jsoup | package org.jsoup.nodes;
import org.jsoup.Jsoup;
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class AttributeTest {
@Test public void html() {
Attribute attr = new Attribute("key", "value &");
assertEquals("key=\"value &\"", attr.html());
assertEquals(attr.html(), attr.toString());
}
@Test public void testWithSupplementaryCharacterInAttributeKeyAndValue() {
String s = new String(Character.toChars(135361));
Attribute attr = new Attribute(s, "A" + s + "B");
assertEquals(s + "=\"A" + s + "B\"", attr.html());
assertEquals(attr.html(), attr.toString());
}
@Test(expected = IllegalArgumentException.class) public void validatesKeysNotEmpty() {
Attribute attr = new Attribute(" ", "Check");
}
@Test(expected = IllegalArgumentException.class) public void validatesKeysNotEmptyViaSet() {
Attribute attr = new Attribute("One", "Check");
attr.setKey(" ");
}
@Test public void booleanAttributesAreEmptyStringValues() {
Document doc = Jsoup.parse("<div hidden>");
Attributes attributes = doc.body().child(0).attributes();
assertEquals("", attributes.get("hidden"));
Attribute first = attributes.iterator().next();
assertEquals("hidden", first.getKey());
assertEquals("", first.getValue());
}
@Test public void settersOnOrphanAttribute() {
Attribute attr = new Attribute("one", "two");
attr.setKey("three");
String oldVal = attr.setValue("four");
assertEquals("two", oldVal);
assertEquals("three", attr.getKey());
assertEquals("four", attr.getValue());
assertEquals(null, attr.parent);
}
} |
||
public void testRecordInference() {
inFunction(
"/** @param {{a: (boolean|undefined)}|{b: (string|undefined)}} x */" +
"function f(x) {}" +
"var out = {};" +
"f(out);");
assertEquals("{a: (boolean|undefined), b: (string|undefined)}",
getType("out").toString());
} | com.google.javascript.jscomp.TypeInferenceTest::testRecordInference | test/com/google/javascript/jscomp/TypeInferenceTest.java | 1,014 | test/com/google/javascript/jscomp/TypeInferenceTest.java | testRecordInference | /*
* Copyright 2008 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import static com.google.javascript.rhino.jstype.JSTypeNative.ALL_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.ARRAY_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.BOOLEAN_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.CHECKED_UNKNOWN_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.FUNCTION_INSTANCE_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.NULL_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.NUMBER_OBJECT_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.NUMBER_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.OBJECT_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.STRING_OBJECT_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.STRING_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.UNKNOWN_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.VOID_TYPE;
import com.google.common.base.Joiner;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.Maps;
import com.google.javascript.jscomp.CodingConvention.AssertionFunctionSpec;
import com.google.javascript.jscomp.CompilerOptions.LanguageMode;
import com.google.javascript.jscomp.DataFlowAnalysis.BranchedFlowState;
import com.google.javascript.jscomp.type.FlowScope;
import com.google.javascript.jscomp.type.ReverseAbstractInterpreter;
import com.google.javascript.rhino.Node;
import com.google.javascript.rhino.jstype.EnumType;
import com.google.javascript.rhino.jstype.JSType;
import com.google.javascript.rhino.jstype.JSTypeNative;
import com.google.javascript.rhino.jstype.JSTypeRegistry;
import com.google.javascript.rhino.jstype.ObjectType;
import com.google.javascript.rhino.jstype.StaticSlot;
import com.google.javascript.rhino.testing.Asserts;
import junit.framework.TestCase;
import java.util.Map;
/**
* Tests {@link TypeInference}.
*
*/
public class TypeInferenceTest extends TestCase {
private Compiler compiler;
private JSTypeRegistry registry;
private Map<String, JSType> assumptions;
private JSType assumedThisType;
private FlowScope returnScope;
private static final Map<String, AssertionFunctionSpec>
ASSERTION_FUNCTION_MAP = Maps.newHashMap();
static {
for (AssertionFunctionSpec func :
new ClosureCodingConvention().getAssertionFunctions()) {
ASSERTION_FUNCTION_MAP.put(func.getFunctionName(), func);
}
}
@Override
public void setUp() {
compiler = new Compiler();
CompilerOptions options = new CompilerOptions();
options.setClosurePass(true);
options.setLanguageIn(LanguageMode.ECMASCRIPT5);
compiler.initOptions(options);
registry = compiler.getTypeRegistry();
assumptions = Maps.newHashMap();
returnScope = null;
}
private void assumingThisType(JSType type) {
assumedThisType = type;
}
private void assuming(String name, JSType type) {
assumptions.put(name, type);
}
private void assuming(String name, JSTypeNative type) {
assuming(name, registry.getNativeType(type));
}
private void inFunction(String js) {
// Parse the body of the function.
String thisBlock = assumedThisType == null
? ""
: "/** @this {" + assumedThisType + "} */";
Node root = compiler.parseTestCode(
"(" + thisBlock + " function() {" + js + "});");
assertEquals("parsing error: " +
Joiner.on(", ").join(compiler.getErrors()),
0, compiler.getErrorCount());
Node n = root.getFirstChild().getFirstChild();
// Create the scope with the assumptions.
TypedScopeCreator scopeCreator = new TypedScopeCreator(compiler);
Scope assumedScope = scopeCreator.createScope(
n, scopeCreator.createScope(root, null));
for (Map.Entry<String,JSType> entry : assumptions.entrySet()) {
assumedScope.declare(entry.getKey(), null, entry.getValue(), null, false);
}
// Create the control graph.
ControlFlowAnalysis cfa = new ControlFlowAnalysis(compiler, false, false);
cfa.process(null, n);
ControlFlowGraph<Node> cfg = cfa.getCfg();
// Create a simple reverse abstract interpreter.
ReverseAbstractInterpreter rai = compiler.getReverseAbstractInterpreter();
// Do the type inference by data-flow analysis.
TypeInference dfa = new TypeInference(compiler, cfg, rai, assumedScope,
ASSERTION_FUNCTION_MAP);
dfa.analyze();
// Get the scope of the implicit return.
BranchedFlowState<FlowScope> rtnState =
cfg.getImplicitReturn().getAnnotation();
returnScope = rtnState.getIn();
}
private JSType getType(String name) {
assertTrue("The return scope should not be null.", returnScope != null);
StaticSlot<JSType> var = returnScope.getSlot(name);
assertTrue("The variable " + name + " is missing from the scope.",
var != null);
return var.getType();
}
private void verify(String name, JSType type) {
Asserts.assertTypeEquals(type, getType(name));
}
private void verify(String name, JSTypeNative type) {
verify(name, registry.getNativeType(type));
}
private void verifySubtypeOf(String name, JSType type) {
JSType varType = getType(name);
assertTrue("The variable " + name + " is missing a type.", varType != null);
assertTrue("The type " + varType + " of variable " + name +
" is not a subtype of " + type +".", varType.isSubtype(type));
}
private void verifySubtypeOf(String name, JSTypeNative type) {
verifySubtypeOf(name, registry.getNativeType(type));
}
private EnumType createEnumType(String name, JSTypeNative elemType) {
return createEnumType(name, registry.getNativeType(elemType));
}
private EnumType createEnumType(String name, JSType elemType) {
return registry.createEnumType(name, null, elemType);
}
private JSType createUndefinableType(JSTypeNative type) {
return registry.createUnionType(
registry.getNativeType(type), registry.getNativeType(VOID_TYPE));
}
private JSType createNullableType(JSTypeNative type) {
return createNullableType(registry.getNativeType(type));
}
private JSType createNullableType(JSType type) {
return registry.createNullableType(type);
}
private JSType createUnionType(JSTypeNative type1, JSTypeNative type2) {
return registry.createUnionType(
registry.getNativeType(type1), registry.getNativeType(type2));
}
public void testAssumption() {
assuming("x", NUMBER_TYPE);
inFunction("");
verify("x", NUMBER_TYPE);
}
public void testVar() {
inFunction("var x = 1;");
verify("x", NUMBER_TYPE);
}
public void testEmptyVar() {
inFunction("var x;");
verify("x", VOID_TYPE);
}
public void testAssignment() {
assuming("x", OBJECT_TYPE);
inFunction("x = 1;");
verify("x", NUMBER_TYPE);
}
public void testGetProp() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("x.y();");
verify("x", OBJECT_TYPE);
}
public void testGetElemDereference() {
assuming("x", createUndefinableType(OBJECT_TYPE));
inFunction("x['z'] = 3;");
verify("x", OBJECT_TYPE);
}
public void testIf1() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = {}; if (x) { y = x; }");
verifySubtypeOf("y", OBJECT_TYPE);
}
public void testIf1a() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = {}; if (x != null) { y = x; }");
verifySubtypeOf("y", OBJECT_TYPE);
}
public void testIf2() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = x; if (x) { y = x; } else { y = {}; }");
verifySubtypeOf("y", OBJECT_TYPE);
}
public void testIf3() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = 1; if (x) { y = x; }");
verify("y", createUnionType(OBJECT_TYPE, NUMBER_TYPE));
}
public void testPropertyInference1() {
ObjectType thisType = registry.createAnonymousObjectType();
thisType.defineDeclaredProperty("foo",
createUndefinableType(STRING_TYPE), null);
assumingThisType(thisType);
inFunction("var y = 1; if (this.foo) { y = this.foo; }");
verify("y", createUnionType(NUMBER_TYPE, STRING_TYPE));
}
public void testPropertyInference2() {
ObjectType thisType = registry.createAnonymousObjectType();
thisType.defineDeclaredProperty("foo",
createUndefinableType(STRING_TYPE), null);
assumingThisType(thisType);
inFunction("var y = 1; this.foo = 'x'; y = this.foo;");
verify("y", STRING_TYPE);
}
public void testPropertyInference3() {
ObjectType thisType = registry.createAnonymousObjectType();
thisType.defineDeclaredProperty("foo",
createUndefinableType(STRING_TYPE), null);
assumingThisType(thisType);
inFunction("var y = 1; this.foo = x; y = this.foo;");
verify("y", CHECKED_UNKNOWN_TYPE);
}
public void testAssert1() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assert(x); out2 = x;");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
}
public void testAssert1a() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assert(x !== null); out2 = x;");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
}
public void testAssert2() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
inFunction("goog.asserts.assert(1, x); out1 = x;");
verify("out1", startType);
}
public void testAssert3() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
assuming("y", startType);
inFunction("out1 = x; goog.asserts.assert(x && y); out2 = x; out3 = y;");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
verify("out3", OBJECT_TYPE);
}
public void testAssert4() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
assuming("y", startType);
inFunction("out1 = x; goog.asserts.assert(x && !y); out2 = x; out3 = y;");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
verify("out3", NULL_TYPE);
}
public void testAssert5() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
assuming("y", startType);
inFunction("goog.asserts.assert(x || y); out1 = x; out2 = y;");
verify("out1", startType);
verify("out2", startType);
}
public void testAssert6() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x.y", startType);
inFunction("out1 = x.y; goog.asserts.assert(x.y); out2 = x.y;");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
}
public void testAssert7() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
inFunction("out1 = x; out2 = goog.asserts.assert(x);");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
}
public void testAssert8() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
inFunction("out1 = x; out2 = goog.asserts.assert(x != null);");
verify("out1", startType);
verify("out2", BOOLEAN_TYPE);
}
public void testAssert9() {
JSType startType = createNullableType(NUMBER_TYPE);
assuming("x", startType);
inFunction("out1 = x; out2 = goog.asserts.assert(y = x);");
verify("out1", startType);
verify("out2", NUMBER_TYPE);
}
public void testAssert10() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
assuming("y", startType);
inFunction("out1 = x; out2 = goog.asserts.assert(x && y); out3 = x;");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
verify("out3", OBJECT_TYPE);
}
public void testAssertNumber() {
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertNumber(x); out2 = x;");
verify("out1", startType);
verify("out2", NUMBER_TYPE);
}
public void testAssertNumber2() {
// Make sure it ignores expressions.
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("goog.asserts.assertNumber(x + x); out1 = x;");
verify("out1", startType);
}
public void testAssertNumber3() {
// Make sure it ignores expressions.
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("out1 = x; out2 = goog.asserts.assertNumber(x + x);");
verify("out1", startType);
verify("out2", NUMBER_TYPE);
}
public void testAssertString() {
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertString(x); out2 = x;");
verify("out1", startType);
verify("out2", STRING_TYPE);
}
public void testAssertFunction() {
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertFunction(x); out2 = x;");
verify("out1", startType);
verifySubtypeOf("out2", FUNCTION_INSTANCE_TYPE);
}
public void testAssertObject() {
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertObject(x); out2 = x;");
verify("out1", startType);
verifySubtypeOf("out2", OBJECT_TYPE);
}
public void testAssertObject2() {
JSType startType = createNullableType(ARRAY_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertObject(x); out2 = x;");
verify("out1", startType);
verify("out2", ARRAY_TYPE);
}
public void testAssertObject3() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x.y", startType);
inFunction("out1 = x.y; goog.asserts.assertObject(x.y); out2 = x.y;");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
}
public void testAssertObject4() {
JSType startType = createNullableType(ARRAY_TYPE);
assuming("x", startType);
inFunction("out1 = x; out2 = goog.asserts.assertObject(x);");
verify("out1", startType);
verify("out2", ARRAY_TYPE);
}
public void testAssertObject5() {
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction(
"out1 = x;" +
"out2 = /** @type {!Array} */ (goog.asserts.assertObject(x));");
verify("out1", startType);
verify("out2", ARRAY_TYPE);
}
public void testAssertArray() {
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertArray(x); out2 = x;");
verify("out1", startType);
verifySubtypeOf("out2", ARRAY_TYPE);
}
public void testAssertInstanceof1() {
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertInstanceof(x); out2 = x;");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
}
public void testAssertInstanceof2() {
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertInstanceof(x, String); out2 = x;");
verify("out1", startType);
verify("out2", STRING_OBJECT_TYPE);
}
public void testAssertInstanceof3() {
JSType startType = registry.getNativeType(UNKNOWN_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertInstanceof(x, String); out2 = x;");
verify("out1", startType);
verify("out2", UNKNOWN_TYPE);
}
public void testAssertInstanceof4() {
JSType startType = registry.getNativeType(STRING_OBJECT_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertInstanceof(x, Object); out2 = x;");
verify("out1", startType);
verify("out2", STRING_OBJECT_TYPE);
}
public void testAssertInstanceof5() {
JSType startType = registry.getNativeType(ALL_TYPE);
assuming("x", startType);
inFunction(
"out1 = x; goog.asserts.assertInstanceof(x, String); var r = x;");
verify("out1", startType);
verify("x", STRING_OBJECT_TYPE);
}
public void testAssertWithIsDef() {
JSType startType = createNullableType(NUMBER_TYPE);
assuming("x", startType);
inFunction(
"out1 = x;" +
"goog.asserts.assert(goog.isDefAndNotNull(x));" +
"out2 = x;");
verify("out1", startType);
verify("out2", NUMBER_TYPE);
}
public void testAssertWithNotIsNull() {
JSType startType = createNullableType(NUMBER_TYPE);
assuming("x", startType);
inFunction(
"out1 = x;" +
"goog.asserts.assert(!goog.isNull(x));" +
"out2 = x;");
verify("out1", startType);
verify("out2", NUMBER_TYPE);
}
public void testReturn1() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("if (x) { return x; }\nx = {};\nreturn x;");
verify("x", OBJECT_TYPE);
}
public void testReturn2() {
assuming("x", createNullableType(NUMBER_TYPE));
inFunction("if (!x) { x = 0; }\nreturn x;");
verify("x", NUMBER_TYPE);
}
public void testWhile1() {
assuming("x", createNullableType(NUMBER_TYPE));
inFunction("while (!x) { if (x == null) { x = 0; } else { x = 1; } }");
verify("x", NUMBER_TYPE);
}
public void testWhile2() {
assuming("x", createNullableType(NUMBER_TYPE));
inFunction("while (!x) { x = {}; }");
verifySubtypeOf("x", createUnionType(OBJECT_TYPE, NUMBER_TYPE));
}
public void testDo() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("do { x = 1; } while (!x);");
verify("x", NUMBER_TYPE);
}
public void testFor1() {
assuming("y", NUMBER_TYPE);
inFunction("var x = null; var i = null; for (i=y; !i; i=1) { x = 1; }");
verify("x", createNullableType(NUMBER_TYPE));
verify("i", NUMBER_TYPE);
}
public void testFor2() {
assuming("y", OBJECT_TYPE);
inFunction("var x = null; var i = null; for (i in y) { x = 1; }");
verify("x", createNullableType(NUMBER_TYPE));
verify("i", createNullableType(STRING_TYPE));
}
public void testFor3() {
assuming("y", OBJECT_TYPE);
inFunction("var x = null; var i = null; for (var i in y) { x = 1; }");
verify("x", createNullableType(NUMBER_TYPE));
verify("i", createNullableType(STRING_TYPE));
}
public void testFor4() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = {};\n" +
"if (x) { for (var i = 0; i < 10; i++) { break; } y = x; }");
verifySubtypeOf("y", OBJECT_TYPE);
}
public void testSwitch1() {
assuming("x", NUMBER_TYPE);
inFunction("var y = null; switch(x) {\n" +
"case 1: y = 1; break;\n" +
"case 2: y = {};\n" +
"case 3: y = {};\n" +
"default: y = 0;}");
verify("y", NUMBER_TYPE);
}
public void testSwitch2() {
assuming("x", ALL_TYPE);
inFunction("var y = null; switch (typeof x) {\n" +
"case 'string':\n" +
" y = x;\n" +
" return;" +
"default:\n" +
" y = 'a';\n" +
"}");
verify("y", STRING_TYPE);
}
public void testSwitch3() {
assuming("x",
createNullableType(createUnionType(NUMBER_TYPE, STRING_TYPE)));
inFunction("var y; var z; switch (typeof x) {\n" +
"case 'string':\n" +
" y = 1; z = null;\n" +
" return;\n" +
"case 'number':\n" +
" y = x; z = null;\n" +
" return;" +
"default:\n" +
" y = 1; z = x;\n" +
"}");
verify("y", NUMBER_TYPE);
verify("z", NULL_TYPE);
}
public void testSwitch4() {
assuming("x", ALL_TYPE);
inFunction("var y = null; switch (typeof x) {\n" +
"case 'string':\n" +
"case 'number':\n" +
" y = x;\n" +
" return;\n" +
"default:\n" +
" y = 1;\n" +
"}\n");
verify("y", createUnionType(NUMBER_TYPE, STRING_TYPE));
}
public void testCall1() {
assuming("x",
createNullableType(
registry.createFunctionType(registry.getNativeType(NUMBER_TYPE))));
inFunction("var y = x();");
verify("y", NUMBER_TYPE);
}
public void testNew1() {
assuming("x",
createNullableType(
registry.getNativeType(JSTypeNative.U2U_CONSTRUCTOR_TYPE)));
inFunction("var y = new x();");
verify("y", JSTypeNative.NO_OBJECT_TYPE);
}
public void testInnerFunction1() {
inFunction("var x = 1; function f() { x = null; };");
verify("x", NUMBER_TYPE);
}
public void testInnerFunction2() {
inFunction("var x = 1; var f = function() { x = null; };");
verify("x", NUMBER_TYPE);
}
public void testHook() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = x ? x : {};");
verifySubtypeOf("y", OBJECT_TYPE);
}
public void testThrow() {
assuming("x", createNullableType(NUMBER_TYPE));
inFunction("var y = 1;\n" +
"if (x == null) { throw new Error('x is null') }\n" +
"y = x;");
verify("y", NUMBER_TYPE);
}
public void testTry1() {
assuming("x", NUMBER_TYPE);
inFunction("var y = null; try { y = null; } finally { y = x; }");
verify("y", NUMBER_TYPE);
}
public void testTry2() {
assuming("x", NUMBER_TYPE);
inFunction("var y = null;\n" +
"try { } catch (e) { y = null; } finally { y = x; }");
verify("y", NUMBER_TYPE);
}
public void testTry3() {
assuming("x", NUMBER_TYPE);
inFunction("var y = null; try { y = x; } catch (e) { }");
verify("y", NUMBER_TYPE);
}
public void testCatch1() {
inFunction("var y = null; try { foo(); } catch (e) { y = e; }");
verify("y", UNKNOWN_TYPE);
}
public void testCatch2() {
inFunction("var y = null; var e = 3; try { foo(); } catch (e) { y = e; }");
verify("y", UNKNOWN_TYPE);
}
public void testUnknownType1() {
inFunction("var y = 3; y = x;");
verify("y", UNKNOWN_TYPE);
}
public void testUnknownType2() {
assuming("x", ARRAY_TYPE);
inFunction("var y = 5; y = x[0];");
verify("y", UNKNOWN_TYPE);
}
public void testInfiniteLoop1() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("x = {}; while(x != null) { x = {}; }");
}
public void testInfiniteLoop2() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("x = {}; do { x = null; } while (x == null);");
}
public void testJoin1() {
JSType unknownOrNull = createUnionType(NULL_TYPE, UNKNOWN_TYPE);
assuming("x", BOOLEAN_TYPE);
assuming("unknownOrNull", unknownOrNull);
inFunction("var y; if (x) y = unknownOrNull; else y = null;");
verify("y", unknownOrNull);
}
public void testJoin2() {
JSType unknownOrNull = createUnionType(NULL_TYPE, UNKNOWN_TYPE);
assuming("x", BOOLEAN_TYPE);
assuming("unknownOrNull", unknownOrNull);
inFunction("var y; if (x) y = null; else y = unknownOrNull;");
verify("y", unknownOrNull);
}
public void testArrayLit() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = 3; if (x) { x = [y = x]; }");
verify("x", createUnionType(NULL_TYPE, ARRAY_TYPE));
verify("y", createUnionType(NUMBER_TYPE, OBJECT_TYPE));
}
public void testGetElem() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = 3; if (x) { x = x[y = x]; }");
verify("x", UNKNOWN_TYPE);
verify("y", createUnionType(NUMBER_TYPE, OBJECT_TYPE));
}
public void testEnumRAI1() {
JSType enumType = createEnumType("MyEnum", ARRAY_TYPE).getElementsType();
assuming("x", enumType);
inFunction("var y = null; if (x) y = x;");
verify("y", createNullableType(enumType));
}
public void testEnumRAI2() {
JSType enumType = createEnumType("MyEnum", NUMBER_TYPE).getElementsType();
assuming("x", enumType);
inFunction("var y = null; if (typeof x == 'number') y = x;");
verify("y", createNullableType(enumType));
}
public void testEnumRAI3() {
JSType enumType = createEnumType("MyEnum", NUMBER_TYPE).getElementsType();
assuming("x", enumType);
inFunction("var y = null; if (x && typeof x == 'number') y = x;");
verify("y", createNullableType(enumType));
}
public void testEnumRAI4() {
JSType enumType = createEnumType("MyEnum",
createUnionType(STRING_TYPE, NUMBER_TYPE)).getElementsType();
assuming("x", enumType);
inFunction("var y = null; if (typeof x == 'number') y = x;");
verify("y", createNullableType(NUMBER_TYPE));
}
public void testShortCircuitingAnd() {
assuming("x", NUMBER_TYPE);
inFunction("var y = null; if (x && (y = 3)) { }");
verify("y", createNullableType(NUMBER_TYPE));
}
public void testShortCircuitingAnd2() {
assuming("x", NUMBER_TYPE);
inFunction("var y = null; var z = 4; if (x && (y = 3)) { z = y; }");
verify("z", NUMBER_TYPE);
}
public void testShortCircuitingOr() {
assuming("x", NUMBER_TYPE);
inFunction("var y = null; if (x || (y = 3)) { }");
verify("y", createNullableType(NUMBER_TYPE));
}
public void testShortCircuitingOr2() {
assuming("x", NUMBER_TYPE);
inFunction("var y = null; var z = 4; if (x || (y = 3)) { z = y; }");
verify("z", createNullableType(NUMBER_TYPE));
}
public void testAssignInCondition() {
assuming("x", createNullableType(NUMBER_TYPE));
inFunction("var y; if (!(y = x)) { y = 3; }");
verify("y", NUMBER_TYPE);
}
public void testInstanceOf1() {
assuming("x", OBJECT_TYPE);
inFunction("var y = null; if (x instanceof String) y = x;");
verify("y", createNullableType(STRING_OBJECT_TYPE));
}
public void testInstanceOf2() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = 1; if (x instanceof String) y = x;");
verify("y", createUnionType(STRING_OBJECT_TYPE, NUMBER_TYPE));
}
public void testInstanceOf3() {
assuming("x", createUnionType(STRING_OBJECT_TYPE, NUMBER_OBJECT_TYPE));
inFunction("var y = null; if (x instanceof String) y = x;");
verify("y", createNullableType(STRING_OBJECT_TYPE));
}
public void testInstanceOf4() {
assuming("x", createUnionType(STRING_OBJECT_TYPE, NUMBER_OBJECT_TYPE));
inFunction("var y = null; if (x instanceof String); else y = x;");
verify("y", createNullableType(NUMBER_OBJECT_TYPE));
}
public void testInstanceOf5() {
assuming("x", OBJECT_TYPE);
inFunction("var y = null; if (x instanceof String); else y = x;");
verify("y", createNullableType(OBJECT_TYPE));
}
public void testInstanceOf6() {
// Here we are using "instanceof" to restrict the unknown type to
// the type of the instance. This has the following problems:
// 1) The type may actually be any sub-type
// 2) The type may implement any interface
// After the instanceof we will require casts for methods that require
// sub-type or unrelated interfaces which would not have been required
// before.
JSType startType = registry.getNativeType(UNKNOWN_TYPE);
assuming("x", startType);
inFunction("out1 = x; if (x instanceof String) out2 = x;");
verify("out1", startType);
verify("out2", STRING_OBJECT_TYPE);
}
public void testFlattening() {
for (int i = 0; i < LinkedFlowScope.MAX_DEPTH + 1; i++) {
assuming("s" + i, ALL_TYPE);
}
assuming("b", JSTypeNative.BOOLEAN_TYPE);
StringBuilder body = new StringBuilder();
body.append("if (b) {");
for (int i = 0; i < LinkedFlowScope.MAX_DEPTH + 1; i++) {
body.append("s");
body.append(i);
body.append(" = 1;\n");
}
body.append(" } else { ");
for (int i = 0; i < LinkedFlowScope.MAX_DEPTH + 1; i++) {
body.append("s");
body.append(i);
body.append(" = 'ONE';\n");
}
body.append("}");
JSType numberORString = createUnionType(NUMBER_TYPE, STRING_TYPE);
inFunction(body.toString());
for (int i = 0; i < LinkedFlowScope.MAX_DEPTH + 1; i++) {
verify("s" + i, numberORString);
}
}
public void testUnary() {
assuming("x", NUMBER_TYPE);
inFunction("var y = +x;");
verify("y", NUMBER_TYPE);
inFunction("var z = -x;");
verify("z", NUMBER_TYPE);
}
public void testAdd1() {
assuming("x", NUMBER_TYPE);
inFunction("var y = x + 5;");
verify("y", NUMBER_TYPE);
}
public void testAdd2() {
assuming("x", NUMBER_TYPE);
inFunction("var y = x + '5';");
verify("y", STRING_TYPE);
}
public void testAdd3() {
assuming("x", NUMBER_TYPE);
inFunction("var y = '5' + x;");
verify("y", STRING_TYPE);
}
public void testAssignAdd() {
assuming("x", NUMBER_TYPE);
inFunction("x += '5';");
verify("x", STRING_TYPE);
}
public void testComparison() {
inFunction("var x = 'foo'; var y = (x = 3) < 4;");
verify("x", NUMBER_TYPE);
inFunction("var x = 'foo'; var y = (x = 3) > 4;");
verify("x", NUMBER_TYPE);
inFunction("var x = 'foo'; var y = (x = 3) <= 4;");
verify("x", NUMBER_TYPE);
inFunction("var x = 'foo'; var y = (x = 3) >= 4;");
verify("x", NUMBER_TYPE);
}
public void testThrownExpression() {
inFunction("var x = 'foo'; "
+ "try { throw new Error(x = 3); } catch (ex) {}");
verify("x", NUMBER_TYPE);
}
public void testObjectLit() {
inFunction("var x = {}; var out = x.a;");
verify("out", UNKNOWN_TYPE); // Shouldn't this be 'undefined'?
inFunction("var x = {a:1}; var out = x.a;");
verify("out", NUMBER_TYPE);
inFunction("var x = {a:1}; var out = x.a; x.a = 'string'; var out2 = x.a;");
verify("out", NUMBER_TYPE);
verify("out2", STRING_TYPE);
inFunction("var x = { get a() {return 1} }; var out = x.a;");
verify("out", UNKNOWN_TYPE);
inFunction(
"var x = {" +
" /** @return {number} */ get a() {return 1}" +
"};" +
"var out = x.a;");
verify("out", NUMBER_TYPE);
inFunction("var x = { set a(b) {} }; var out = x.a;");
verify("out", UNKNOWN_TYPE);
inFunction("var x = { " +
"/** @param {number} b */ set a(b) {} };" +
"var out = x.a;");
verify("out", NUMBER_TYPE);
}
public void testCast1() {
inFunction("var x = /** @type {Object} */ (this);");
verify("x", createNullableType(OBJECT_TYPE));
}
public void testCast2() {
inFunction(
"/** @return {boolean} */" +
"Object.prototype.method = function() { return true; };" +
"var x = /** @type {Object} */ (this).method;");
verify(
"x",
registry.createFunctionType(
registry.getNativeObjectType(OBJECT_TYPE),
registry.getNativeType(BOOLEAN_TYPE),
ImmutableList.<JSType>of() /* params */));
}
public void testBackwardsInferenceCall() {
inFunction(
"/** @param {{foo: (number|undefined)}} x */" +
"function f(x) {}" +
"var y = {};" +
"f(y);");
assertEquals("{foo: (number|undefined)}", getType("y").toString());
}
public void testBackwardsInferenceNew() {
inFunction(
"/**\n" +
" * @constructor\n" +
" * @param {{foo: (number|undefined)}} x\n" +
" */" +
"function F(x) {}" +
"var y = {};" +
"new F(y);");
assertEquals("{foo: (number|undefined)}", getType("y").toString());
}
public void testNoThisInference() {
JSType thisType = createNullableType(OBJECT_TYPE);
assumingThisType(thisType);
inFunction("var out = 3; if (goog.isNull(this)) out = this;");
verify("out", createUnionType(OBJECT_TYPE, NUMBER_TYPE));
}
public void testRecordInference() {
inFunction(
"/** @param {{a: (boolean|undefined)}|{b: (string|undefined)}} x */" +
"function f(x) {}" +
"var out = {};" +
"f(out);");
assertEquals("{a: (boolean|undefined), b: (string|undefined)}",
getType("out").toString());
}
public void testIssue785() {
inFunction("/** @param {string|{prop: (string|undefined)}} x */" +
"function f(x) {}" +
"var out = {};" +
"f(out);");
assertEquals("{prop: (string|undefined)}", getType("out").toString());
}
} | // You are a professional Java test case writer, please create a test case named `testRecordInference` for the issue `Closure-785`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-785
//
// ## Issue-Title:
// anonymous object type inference inconsistency when used in union
//
// ## Issue-Description:
// Code:
// /\*\* @param {{prop: string, prop2: (string|undefined)}} record \*/
// var func = function(record) {
// window.console.log(record.prop);
// }
//
// /\*\* @param {{prop: string, prop2: (string|undefined)}|string} record \*/
// var func2 = function(record) {
// if (typeof record == 'string') {
// window.console.log(record);
// } else {
// window.console.log(record.prop);
// }
// }
//
// func({prop: 'a'});
// func2({prop: 'a'});
//
//
//
//
// errors with:
// ERROR - actual parameter 1 of func2 does not match formal parameter
// found : {prop: string}
// required: (string|{prop: string, prop2: (string|undefined)})
// func2({prop: 'a'});
//
//
// the type of the record input to func and func2 are identical but the parameters to func2 allow some other type.
//
//
public void testRecordInference() {
| 1,014 | 166 | 1,006 | test/com/google/javascript/jscomp/TypeInferenceTest.java | test | ```markdown
## Issue-ID: Closure-785
## Issue-Title:
anonymous object type inference inconsistency when used in union
## Issue-Description:
Code:
/\*\* @param {{prop: string, prop2: (string|undefined)}} record \*/
var func = function(record) {
window.console.log(record.prop);
}
/\*\* @param {{prop: string, prop2: (string|undefined)}|string} record \*/
var func2 = function(record) {
if (typeof record == 'string') {
window.console.log(record);
} else {
window.console.log(record.prop);
}
}
func({prop: 'a'});
func2({prop: 'a'});
errors with:
ERROR - actual parameter 1 of func2 does not match formal parameter
found : {prop: string}
required: (string|{prop: string, prop2: (string|undefined)})
func2({prop: 'a'});
the type of the record input to func and func2 are identical but the parameters to func2 allow some other type.
```
You are a professional Java test case writer, please create a test case named `testRecordInference` for the issue `Closure-785`, utilizing the provided issue report information and the following function signature.
```java
public void testRecordInference() {
```
| 1,006 | [
"com.google.javascript.rhino.jstype.PrototypeObjectType"
] | 73378345445725fb540e35473f57fb62be7020020864ea9c90dddc0b1a30f647 | public void testRecordInference() | // You are a professional Java test case writer, please create a test case named `testRecordInference` for the issue `Closure-785`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-785
//
// ## Issue-Title:
// anonymous object type inference inconsistency when used in union
//
// ## Issue-Description:
// Code:
// /\*\* @param {{prop: string, prop2: (string|undefined)}} record \*/
// var func = function(record) {
// window.console.log(record.prop);
// }
//
// /\*\* @param {{prop: string, prop2: (string|undefined)}|string} record \*/
// var func2 = function(record) {
// if (typeof record == 'string') {
// window.console.log(record);
// } else {
// window.console.log(record.prop);
// }
// }
//
// func({prop: 'a'});
// func2({prop: 'a'});
//
//
//
//
// errors with:
// ERROR - actual parameter 1 of func2 does not match formal parameter
// found : {prop: string}
// required: (string|{prop: string, prop2: (string|undefined)})
// func2({prop: 'a'});
//
//
// the type of the record input to func and func2 are identical but the parameters to func2 allow some other type.
//
//
| Closure | /*
* Copyright 2008 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import static com.google.javascript.rhino.jstype.JSTypeNative.ALL_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.ARRAY_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.BOOLEAN_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.CHECKED_UNKNOWN_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.FUNCTION_INSTANCE_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.NULL_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.NUMBER_OBJECT_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.NUMBER_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.OBJECT_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.STRING_OBJECT_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.STRING_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.UNKNOWN_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.VOID_TYPE;
import com.google.common.base.Joiner;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.Maps;
import com.google.javascript.jscomp.CodingConvention.AssertionFunctionSpec;
import com.google.javascript.jscomp.CompilerOptions.LanguageMode;
import com.google.javascript.jscomp.DataFlowAnalysis.BranchedFlowState;
import com.google.javascript.jscomp.type.FlowScope;
import com.google.javascript.jscomp.type.ReverseAbstractInterpreter;
import com.google.javascript.rhino.Node;
import com.google.javascript.rhino.jstype.EnumType;
import com.google.javascript.rhino.jstype.JSType;
import com.google.javascript.rhino.jstype.JSTypeNative;
import com.google.javascript.rhino.jstype.JSTypeRegistry;
import com.google.javascript.rhino.jstype.ObjectType;
import com.google.javascript.rhino.jstype.StaticSlot;
import com.google.javascript.rhino.testing.Asserts;
import junit.framework.TestCase;
import java.util.Map;
/**
* Tests {@link TypeInference}.
*
*/
public class TypeInferenceTest extends TestCase {
private Compiler compiler;
private JSTypeRegistry registry;
private Map<String, JSType> assumptions;
private JSType assumedThisType;
private FlowScope returnScope;
private static final Map<String, AssertionFunctionSpec>
ASSERTION_FUNCTION_MAP = Maps.newHashMap();
static {
for (AssertionFunctionSpec func :
new ClosureCodingConvention().getAssertionFunctions()) {
ASSERTION_FUNCTION_MAP.put(func.getFunctionName(), func);
}
}
@Override
public void setUp() {
compiler = new Compiler();
CompilerOptions options = new CompilerOptions();
options.setClosurePass(true);
options.setLanguageIn(LanguageMode.ECMASCRIPT5);
compiler.initOptions(options);
registry = compiler.getTypeRegistry();
assumptions = Maps.newHashMap();
returnScope = null;
}
private void assumingThisType(JSType type) {
assumedThisType = type;
}
private void assuming(String name, JSType type) {
assumptions.put(name, type);
}
private void assuming(String name, JSTypeNative type) {
assuming(name, registry.getNativeType(type));
}
private void inFunction(String js) {
// Parse the body of the function.
String thisBlock = assumedThisType == null
? ""
: "/** @this {" + assumedThisType + "} */";
Node root = compiler.parseTestCode(
"(" + thisBlock + " function() {" + js + "});");
assertEquals("parsing error: " +
Joiner.on(", ").join(compiler.getErrors()),
0, compiler.getErrorCount());
Node n = root.getFirstChild().getFirstChild();
// Create the scope with the assumptions.
TypedScopeCreator scopeCreator = new TypedScopeCreator(compiler);
Scope assumedScope = scopeCreator.createScope(
n, scopeCreator.createScope(root, null));
for (Map.Entry<String,JSType> entry : assumptions.entrySet()) {
assumedScope.declare(entry.getKey(), null, entry.getValue(), null, false);
}
// Create the control graph.
ControlFlowAnalysis cfa = new ControlFlowAnalysis(compiler, false, false);
cfa.process(null, n);
ControlFlowGraph<Node> cfg = cfa.getCfg();
// Create a simple reverse abstract interpreter.
ReverseAbstractInterpreter rai = compiler.getReverseAbstractInterpreter();
// Do the type inference by data-flow analysis.
TypeInference dfa = new TypeInference(compiler, cfg, rai, assumedScope,
ASSERTION_FUNCTION_MAP);
dfa.analyze();
// Get the scope of the implicit return.
BranchedFlowState<FlowScope> rtnState =
cfg.getImplicitReturn().getAnnotation();
returnScope = rtnState.getIn();
}
private JSType getType(String name) {
assertTrue("The return scope should not be null.", returnScope != null);
StaticSlot<JSType> var = returnScope.getSlot(name);
assertTrue("The variable " + name + " is missing from the scope.",
var != null);
return var.getType();
}
private void verify(String name, JSType type) {
Asserts.assertTypeEquals(type, getType(name));
}
private void verify(String name, JSTypeNative type) {
verify(name, registry.getNativeType(type));
}
private void verifySubtypeOf(String name, JSType type) {
JSType varType = getType(name);
assertTrue("The variable " + name + " is missing a type.", varType != null);
assertTrue("The type " + varType + " of variable " + name +
" is not a subtype of " + type +".", varType.isSubtype(type));
}
private void verifySubtypeOf(String name, JSTypeNative type) {
verifySubtypeOf(name, registry.getNativeType(type));
}
private EnumType createEnumType(String name, JSTypeNative elemType) {
return createEnumType(name, registry.getNativeType(elemType));
}
private EnumType createEnumType(String name, JSType elemType) {
return registry.createEnumType(name, null, elemType);
}
private JSType createUndefinableType(JSTypeNative type) {
return registry.createUnionType(
registry.getNativeType(type), registry.getNativeType(VOID_TYPE));
}
private JSType createNullableType(JSTypeNative type) {
return createNullableType(registry.getNativeType(type));
}
private JSType createNullableType(JSType type) {
return registry.createNullableType(type);
}
private JSType createUnionType(JSTypeNative type1, JSTypeNative type2) {
return registry.createUnionType(
registry.getNativeType(type1), registry.getNativeType(type2));
}
public void testAssumption() {
assuming("x", NUMBER_TYPE);
inFunction("");
verify("x", NUMBER_TYPE);
}
public void testVar() {
inFunction("var x = 1;");
verify("x", NUMBER_TYPE);
}
public void testEmptyVar() {
inFunction("var x;");
verify("x", VOID_TYPE);
}
public void testAssignment() {
assuming("x", OBJECT_TYPE);
inFunction("x = 1;");
verify("x", NUMBER_TYPE);
}
public void testGetProp() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("x.y();");
verify("x", OBJECT_TYPE);
}
public void testGetElemDereference() {
assuming("x", createUndefinableType(OBJECT_TYPE));
inFunction("x['z'] = 3;");
verify("x", OBJECT_TYPE);
}
public void testIf1() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = {}; if (x) { y = x; }");
verifySubtypeOf("y", OBJECT_TYPE);
}
public void testIf1a() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = {}; if (x != null) { y = x; }");
verifySubtypeOf("y", OBJECT_TYPE);
}
public void testIf2() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = x; if (x) { y = x; } else { y = {}; }");
verifySubtypeOf("y", OBJECT_TYPE);
}
public void testIf3() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = 1; if (x) { y = x; }");
verify("y", createUnionType(OBJECT_TYPE, NUMBER_TYPE));
}
public void testPropertyInference1() {
ObjectType thisType = registry.createAnonymousObjectType();
thisType.defineDeclaredProperty("foo",
createUndefinableType(STRING_TYPE), null);
assumingThisType(thisType);
inFunction("var y = 1; if (this.foo) { y = this.foo; }");
verify("y", createUnionType(NUMBER_TYPE, STRING_TYPE));
}
public void testPropertyInference2() {
ObjectType thisType = registry.createAnonymousObjectType();
thisType.defineDeclaredProperty("foo",
createUndefinableType(STRING_TYPE), null);
assumingThisType(thisType);
inFunction("var y = 1; this.foo = 'x'; y = this.foo;");
verify("y", STRING_TYPE);
}
public void testPropertyInference3() {
ObjectType thisType = registry.createAnonymousObjectType();
thisType.defineDeclaredProperty("foo",
createUndefinableType(STRING_TYPE), null);
assumingThisType(thisType);
inFunction("var y = 1; this.foo = x; y = this.foo;");
verify("y", CHECKED_UNKNOWN_TYPE);
}
public void testAssert1() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assert(x); out2 = x;");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
}
public void testAssert1a() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assert(x !== null); out2 = x;");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
}
public void testAssert2() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
inFunction("goog.asserts.assert(1, x); out1 = x;");
verify("out1", startType);
}
public void testAssert3() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
assuming("y", startType);
inFunction("out1 = x; goog.asserts.assert(x && y); out2 = x; out3 = y;");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
verify("out3", OBJECT_TYPE);
}
public void testAssert4() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
assuming("y", startType);
inFunction("out1 = x; goog.asserts.assert(x && !y); out2 = x; out3 = y;");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
verify("out3", NULL_TYPE);
}
public void testAssert5() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
assuming("y", startType);
inFunction("goog.asserts.assert(x || y); out1 = x; out2 = y;");
verify("out1", startType);
verify("out2", startType);
}
public void testAssert6() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x.y", startType);
inFunction("out1 = x.y; goog.asserts.assert(x.y); out2 = x.y;");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
}
public void testAssert7() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
inFunction("out1 = x; out2 = goog.asserts.assert(x);");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
}
public void testAssert8() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
inFunction("out1 = x; out2 = goog.asserts.assert(x != null);");
verify("out1", startType);
verify("out2", BOOLEAN_TYPE);
}
public void testAssert9() {
JSType startType = createNullableType(NUMBER_TYPE);
assuming("x", startType);
inFunction("out1 = x; out2 = goog.asserts.assert(y = x);");
verify("out1", startType);
verify("out2", NUMBER_TYPE);
}
public void testAssert10() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
assuming("y", startType);
inFunction("out1 = x; out2 = goog.asserts.assert(x && y); out3 = x;");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
verify("out3", OBJECT_TYPE);
}
public void testAssertNumber() {
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertNumber(x); out2 = x;");
verify("out1", startType);
verify("out2", NUMBER_TYPE);
}
public void testAssertNumber2() {
// Make sure it ignores expressions.
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("goog.asserts.assertNumber(x + x); out1 = x;");
verify("out1", startType);
}
public void testAssertNumber3() {
// Make sure it ignores expressions.
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("out1 = x; out2 = goog.asserts.assertNumber(x + x);");
verify("out1", startType);
verify("out2", NUMBER_TYPE);
}
public void testAssertString() {
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertString(x); out2 = x;");
verify("out1", startType);
verify("out2", STRING_TYPE);
}
public void testAssertFunction() {
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertFunction(x); out2 = x;");
verify("out1", startType);
verifySubtypeOf("out2", FUNCTION_INSTANCE_TYPE);
}
public void testAssertObject() {
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertObject(x); out2 = x;");
verify("out1", startType);
verifySubtypeOf("out2", OBJECT_TYPE);
}
public void testAssertObject2() {
JSType startType = createNullableType(ARRAY_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertObject(x); out2 = x;");
verify("out1", startType);
verify("out2", ARRAY_TYPE);
}
public void testAssertObject3() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x.y", startType);
inFunction("out1 = x.y; goog.asserts.assertObject(x.y); out2 = x.y;");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
}
public void testAssertObject4() {
JSType startType = createNullableType(ARRAY_TYPE);
assuming("x", startType);
inFunction("out1 = x; out2 = goog.asserts.assertObject(x);");
verify("out1", startType);
verify("out2", ARRAY_TYPE);
}
public void testAssertObject5() {
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction(
"out1 = x;" +
"out2 = /** @type {!Array} */ (goog.asserts.assertObject(x));");
verify("out1", startType);
verify("out2", ARRAY_TYPE);
}
public void testAssertArray() {
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertArray(x); out2 = x;");
verify("out1", startType);
verifySubtypeOf("out2", ARRAY_TYPE);
}
public void testAssertInstanceof1() {
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertInstanceof(x); out2 = x;");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
}
public void testAssertInstanceof2() {
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertInstanceof(x, String); out2 = x;");
verify("out1", startType);
verify("out2", STRING_OBJECT_TYPE);
}
public void testAssertInstanceof3() {
JSType startType = registry.getNativeType(UNKNOWN_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertInstanceof(x, String); out2 = x;");
verify("out1", startType);
verify("out2", UNKNOWN_TYPE);
}
public void testAssertInstanceof4() {
JSType startType = registry.getNativeType(STRING_OBJECT_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertInstanceof(x, Object); out2 = x;");
verify("out1", startType);
verify("out2", STRING_OBJECT_TYPE);
}
public void testAssertInstanceof5() {
JSType startType = registry.getNativeType(ALL_TYPE);
assuming("x", startType);
inFunction(
"out1 = x; goog.asserts.assertInstanceof(x, String); var r = x;");
verify("out1", startType);
verify("x", STRING_OBJECT_TYPE);
}
public void testAssertWithIsDef() {
JSType startType = createNullableType(NUMBER_TYPE);
assuming("x", startType);
inFunction(
"out1 = x;" +
"goog.asserts.assert(goog.isDefAndNotNull(x));" +
"out2 = x;");
verify("out1", startType);
verify("out2", NUMBER_TYPE);
}
public void testAssertWithNotIsNull() {
JSType startType = createNullableType(NUMBER_TYPE);
assuming("x", startType);
inFunction(
"out1 = x;" +
"goog.asserts.assert(!goog.isNull(x));" +
"out2 = x;");
verify("out1", startType);
verify("out2", NUMBER_TYPE);
}
public void testReturn1() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("if (x) { return x; }\nx = {};\nreturn x;");
verify("x", OBJECT_TYPE);
}
public void testReturn2() {
assuming("x", createNullableType(NUMBER_TYPE));
inFunction("if (!x) { x = 0; }\nreturn x;");
verify("x", NUMBER_TYPE);
}
public void testWhile1() {
assuming("x", createNullableType(NUMBER_TYPE));
inFunction("while (!x) { if (x == null) { x = 0; } else { x = 1; } }");
verify("x", NUMBER_TYPE);
}
public void testWhile2() {
assuming("x", createNullableType(NUMBER_TYPE));
inFunction("while (!x) { x = {}; }");
verifySubtypeOf("x", createUnionType(OBJECT_TYPE, NUMBER_TYPE));
}
public void testDo() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("do { x = 1; } while (!x);");
verify("x", NUMBER_TYPE);
}
public void testFor1() {
assuming("y", NUMBER_TYPE);
inFunction("var x = null; var i = null; for (i=y; !i; i=1) { x = 1; }");
verify("x", createNullableType(NUMBER_TYPE));
verify("i", NUMBER_TYPE);
}
public void testFor2() {
assuming("y", OBJECT_TYPE);
inFunction("var x = null; var i = null; for (i in y) { x = 1; }");
verify("x", createNullableType(NUMBER_TYPE));
verify("i", createNullableType(STRING_TYPE));
}
public void testFor3() {
assuming("y", OBJECT_TYPE);
inFunction("var x = null; var i = null; for (var i in y) { x = 1; }");
verify("x", createNullableType(NUMBER_TYPE));
verify("i", createNullableType(STRING_TYPE));
}
public void testFor4() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = {};\n" +
"if (x) { for (var i = 0; i < 10; i++) { break; } y = x; }");
verifySubtypeOf("y", OBJECT_TYPE);
}
public void testSwitch1() {
assuming("x", NUMBER_TYPE);
inFunction("var y = null; switch(x) {\n" +
"case 1: y = 1; break;\n" +
"case 2: y = {};\n" +
"case 3: y = {};\n" +
"default: y = 0;}");
verify("y", NUMBER_TYPE);
}
public void testSwitch2() {
assuming("x", ALL_TYPE);
inFunction("var y = null; switch (typeof x) {\n" +
"case 'string':\n" +
" y = x;\n" +
" return;" +
"default:\n" +
" y = 'a';\n" +
"}");
verify("y", STRING_TYPE);
}
public void testSwitch3() {
assuming("x",
createNullableType(createUnionType(NUMBER_TYPE, STRING_TYPE)));
inFunction("var y; var z; switch (typeof x) {\n" +
"case 'string':\n" +
" y = 1; z = null;\n" +
" return;\n" +
"case 'number':\n" +
" y = x; z = null;\n" +
" return;" +
"default:\n" +
" y = 1; z = x;\n" +
"}");
verify("y", NUMBER_TYPE);
verify("z", NULL_TYPE);
}
public void testSwitch4() {
assuming("x", ALL_TYPE);
inFunction("var y = null; switch (typeof x) {\n" +
"case 'string':\n" +
"case 'number':\n" +
" y = x;\n" +
" return;\n" +
"default:\n" +
" y = 1;\n" +
"}\n");
verify("y", createUnionType(NUMBER_TYPE, STRING_TYPE));
}
public void testCall1() {
assuming("x",
createNullableType(
registry.createFunctionType(registry.getNativeType(NUMBER_TYPE))));
inFunction("var y = x();");
verify("y", NUMBER_TYPE);
}
public void testNew1() {
assuming("x",
createNullableType(
registry.getNativeType(JSTypeNative.U2U_CONSTRUCTOR_TYPE)));
inFunction("var y = new x();");
verify("y", JSTypeNative.NO_OBJECT_TYPE);
}
public void testInnerFunction1() {
inFunction("var x = 1; function f() { x = null; };");
verify("x", NUMBER_TYPE);
}
public void testInnerFunction2() {
inFunction("var x = 1; var f = function() { x = null; };");
verify("x", NUMBER_TYPE);
}
public void testHook() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = x ? x : {};");
verifySubtypeOf("y", OBJECT_TYPE);
}
public void testThrow() {
assuming("x", createNullableType(NUMBER_TYPE));
inFunction("var y = 1;\n" +
"if (x == null) { throw new Error('x is null') }\n" +
"y = x;");
verify("y", NUMBER_TYPE);
}
public void testTry1() {
assuming("x", NUMBER_TYPE);
inFunction("var y = null; try { y = null; } finally { y = x; }");
verify("y", NUMBER_TYPE);
}
public void testTry2() {
assuming("x", NUMBER_TYPE);
inFunction("var y = null;\n" +
"try { } catch (e) { y = null; } finally { y = x; }");
verify("y", NUMBER_TYPE);
}
public void testTry3() {
assuming("x", NUMBER_TYPE);
inFunction("var y = null; try { y = x; } catch (e) { }");
verify("y", NUMBER_TYPE);
}
public void testCatch1() {
inFunction("var y = null; try { foo(); } catch (e) { y = e; }");
verify("y", UNKNOWN_TYPE);
}
public void testCatch2() {
inFunction("var y = null; var e = 3; try { foo(); } catch (e) { y = e; }");
verify("y", UNKNOWN_TYPE);
}
public void testUnknownType1() {
inFunction("var y = 3; y = x;");
verify("y", UNKNOWN_TYPE);
}
public void testUnknownType2() {
assuming("x", ARRAY_TYPE);
inFunction("var y = 5; y = x[0];");
verify("y", UNKNOWN_TYPE);
}
public void testInfiniteLoop1() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("x = {}; while(x != null) { x = {}; }");
}
public void testInfiniteLoop2() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("x = {}; do { x = null; } while (x == null);");
}
public void testJoin1() {
JSType unknownOrNull = createUnionType(NULL_TYPE, UNKNOWN_TYPE);
assuming("x", BOOLEAN_TYPE);
assuming("unknownOrNull", unknownOrNull);
inFunction("var y; if (x) y = unknownOrNull; else y = null;");
verify("y", unknownOrNull);
}
public void testJoin2() {
JSType unknownOrNull = createUnionType(NULL_TYPE, UNKNOWN_TYPE);
assuming("x", BOOLEAN_TYPE);
assuming("unknownOrNull", unknownOrNull);
inFunction("var y; if (x) y = null; else y = unknownOrNull;");
verify("y", unknownOrNull);
}
public void testArrayLit() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = 3; if (x) { x = [y = x]; }");
verify("x", createUnionType(NULL_TYPE, ARRAY_TYPE));
verify("y", createUnionType(NUMBER_TYPE, OBJECT_TYPE));
}
public void testGetElem() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = 3; if (x) { x = x[y = x]; }");
verify("x", UNKNOWN_TYPE);
verify("y", createUnionType(NUMBER_TYPE, OBJECT_TYPE));
}
public void testEnumRAI1() {
JSType enumType = createEnumType("MyEnum", ARRAY_TYPE).getElementsType();
assuming("x", enumType);
inFunction("var y = null; if (x) y = x;");
verify("y", createNullableType(enumType));
}
public void testEnumRAI2() {
JSType enumType = createEnumType("MyEnum", NUMBER_TYPE).getElementsType();
assuming("x", enumType);
inFunction("var y = null; if (typeof x == 'number') y = x;");
verify("y", createNullableType(enumType));
}
public void testEnumRAI3() {
JSType enumType = createEnumType("MyEnum", NUMBER_TYPE).getElementsType();
assuming("x", enumType);
inFunction("var y = null; if (x && typeof x == 'number') y = x;");
verify("y", createNullableType(enumType));
}
public void testEnumRAI4() {
JSType enumType = createEnumType("MyEnum",
createUnionType(STRING_TYPE, NUMBER_TYPE)).getElementsType();
assuming("x", enumType);
inFunction("var y = null; if (typeof x == 'number') y = x;");
verify("y", createNullableType(NUMBER_TYPE));
}
public void testShortCircuitingAnd() {
assuming("x", NUMBER_TYPE);
inFunction("var y = null; if (x && (y = 3)) { }");
verify("y", createNullableType(NUMBER_TYPE));
}
public void testShortCircuitingAnd2() {
assuming("x", NUMBER_TYPE);
inFunction("var y = null; var z = 4; if (x && (y = 3)) { z = y; }");
verify("z", NUMBER_TYPE);
}
public void testShortCircuitingOr() {
assuming("x", NUMBER_TYPE);
inFunction("var y = null; if (x || (y = 3)) { }");
verify("y", createNullableType(NUMBER_TYPE));
}
public void testShortCircuitingOr2() {
assuming("x", NUMBER_TYPE);
inFunction("var y = null; var z = 4; if (x || (y = 3)) { z = y; }");
verify("z", createNullableType(NUMBER_TYPE));
}
public void testAssignInCondition() {
assuming("x", createNullableType(NUMBER_TYPE));
inFunction("var y; if (!(y = x)) { y = 3; }");
verify("y", NUMBER_TYPE);
}
public void testInstanceOf1() {
assuming("x", OBJECT_TYPE);
inFunction("var y = null; if (x instanceof String) y = x;");
verify("y", createNullableType(STRING_OBJECT_TYPE));
}
public void testInstanceOf2() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = 1; if (x instanceof String) y = x;");
verify("y", createUnionType(STRING_OBJECT_TYPE, NUMBER_TYPE));
}
public void testInstanceOf3() {
assuming("x", createUnionType(STRING_OBJECT_TYPE, NUMBER_OBJECT_TYPE));
inFunction("var y = null; if (x instanceof String) y = x;");
verify("y", createNullableType(STRING_OBJECT_TYPE));
}
public void testInstanceOf4() {
assuming("x", createUnionType(STRING_OBJECT_TYPE, NUMBER_OBJECT_TYPE));
inFunction("var y = null; if (x instanceof String); else y = x;");
verify("y", createNullableType(NUMBER_OBJECT_TYPE));
}
public void testInstanceOf5() {
assuming("x", OBJECT_TYPE);
inFunction("var y = null; if (x instanceof String); else y = x;");
verify("y", createNullableType(OBJECT_TYPE));
}
public void testInstanceOf6() {
// Here we are using "instanceof" to restrict the unknown type to
// the type of the instance. This has the following problems:
// 1) The type may actually be any sub-type
// 2) The type may implement any interface
// After the instanceof we will require casts for methods that require
// sub-type or unrelated interfaces which would not have been required
// before.
JSType startType = registry.getNativeType(UNKNOWN_TYPE);
assuming("x", startType);
inFunction("out1 = x; if (x instanceof String) out2 = x;");
verify("out1", startType);
verify("out2", STRING_OBJECT_TYPE);
}
public void testFlattening() {
for (int i = 0; i < LinkedFlowScope.MAX_DEPTH + 1; i++) {
assuming("s" + i, ALL_TYPE);
}
assuming("b", JSTypeNative.BOOLEAN_TYPE);
StringBuilder body = new StringBuilder();
body.append("if (b) {");
for (int i = 0; i < LinkedFlowScope.MAX_DEPTH + 1; i++) {
body.append("s");
body.append(i);
body.append(" = 1;\n");
}
body.append(" } else { ");
for (int i = 0; i < LinkedFlowScope.MAX_DEPTH + 1; i++) {
body.append("s");
body.append(i);
body.append(" = 'ONE';\n");
}
body.append("}");
JSType numberORString = createUnionType(NUMBER_TYPE, STRING_TYPE);
inFunction(body.toString());
for (int i = 0; i < LinkedFlowScope.MAX_DEPTH + 1; i++) {
verify("s" + i, numberORString);
}
}
public void testUnary() {
assuming("x", NUMBER_TYPE);
inFunction("var y = +x;");
verify("y", NUMBER_TYPE);
inFunction("var z = -x;");
verify("z", NUMBER_TYPE);
}
public void testAdd1() {
assuming("x", NUMBER_TYPE);
inFunction("var y = x + 5;");
verify("y", NUMBER_TYPE);
}
public void testAdd2() {
assuming("x", NUMBER_TYPE);
inFunction("var y = x + '5';");
verify("y", STRING_TYPE);
}
public void testAdd3() {
assuming("x", NUMBER_TYPE);
inFunction("var y = '5' + x;");
verify("y", STRING_TYPE);
}
public void testAssignAdd() {
assuming("x", NUMBER_TYPE);
inFunction("x += '5';");
verify("x", STRING_TYPE);
}
public void testComparison() {
inFunction("var x = 'foo'; var y = (x = 3) < 4;");
verify("x", NUMBER_TYPE);
inFunction("var x = 'foo'; var y = (x = 3) > 4;");
verify("x", NUMBER_TYPE);
inFunction("var x = 'foo'; var y = (x = 3) <= 4;");
verify("x", NUMBER_TYPE);
inFunction("var x = 'foo'; var y = (x = 3) >= 4;");
verify("x", NUMBER_TYPE);
}
public void testThrownExpression() {
inFunction("var x = 'foo'; "
+ "try { throw new Error(x = 3); } catch (ex) {}");
verify("x", NUMBER_TYPE);
}
public void testObjectLit() {
inFunction("var x = {}; var out = x.a;");
verify("out", UNKNOWN_TYPE); // Shouldn't this be 'undefined'?
inFunction("var x = {a:1}; var out = x.a;");
verify("out", NUMBER_TYPE);
inFunction("var x = {a:1}; var out = x.a; x.a = 'string'; var out2 = x.a;");
verify("out", NUMBER_TYPE);
verify("out2", STRING_TYPE);
inFunction("var x = { get a() {return 1} }; var out = x.a;");
verify("out", UNKNOWN_TYPE);
inFunction(
"var x = {" +
" /** @return {number} */ get a() {return 1}" +
"};" +
"var out = x.a;");
verify("out", NUMBER_TYPE);
inFunction("var x = { set a(b) {} }; var out = x.a;");
verify("out", UNKNOWN_TYPE);
inFunction("var x = { " +
"/** @param {number} b */ set a(b) {} };" +
"var out = x.a;");
verify("out", NUMBER_TYPE);
}
public void testCast1() {
inFunction("var x = /** @type {Object} */ (this);");
verify("x", createNullableType(OBJECT_TYPE));
}
public void testCast2() {
inFunction(
"/** @return {boolean} */" +
"Object.prototype.method = function() { return true; };" +
"var x = /** @type {Object} */ (this).method;");
verify(
"x",
registry.createFunctionType(
registry.getNativeObjectType(OBJECT_TYPE),
registry.getNativeType(BOOLEAN_TYPE),
ImmutableList.<JSType>of() /* params */));
}
public void testBackwardsInferenceCall() {
inFunction(
"/** @param {{foo: (number|undefined)}} x */" +
"function f(x) {}" +
"var y = {};" +
"f(y);");
assertEquals("{foo: (number|undefined)}", getType("y").toString());
}
public void testBackwardsInferenceNew() {
inFunction(
"/**\n" +
" * @constructor\n" +
" * @param {{foo: (number|undefined)}} x\n" +
" */" +
"function F(x) {}" +
"var y = {};" +
"new F(y);");
assertEquals("{foo: (number|undefined)}", getType("y").toString());
}
public void testNoThisInference() {
JSType thisType = createNullableType(OBJECT_TYPE);
assumingThisType(thisType);
inFunction("var out = 3; if (goog.isNull(this)) out = this;");
verify("out", createUnionType(OBJECT_TYPE, NUMBER_TYPE));
}
public void testRecordInference() {
inFunction(
"/** @param {{a: (boolean|undefined)}|{b: (string|undefined)}} x */" +
"function f(x) {}" +
"var out = {};" +
"f(out);");
assertEquals("{a: (boolean|undefined), b: (string|undefined)}",
getType("out").toString());
}
public void testIssue785() {
inFunction("/** @param {string|{prop: (string|undefined)}} x */" +
"function f(x) {}" +
"var out = {};" +
"f(out);");
assertEquals("{prop: (string|undefined)}", getType("out").toString());
}
} |
||
public void testDateDeserializationISO8601() throws Exception {
DefaultDateTypeAdapter adapter = new DefaultDateTypeAdapter();
assertParsed("1970-01-01T00:00:00.000Z", adapter);
assertParsed("1970-01-01T00:00Z", adapter);
assertParsed("1970-01-01T00:00:00+00:00", adapter);
assertParsed("1970-01-01T01:00:00+01:00", adapter);
assertParsed("1970-01-01T01:00:00+01", adapter);
} | com.google.gson.DefaultDateTypeAdapterTest::testDateDeserializationISO8601 | gson/src/test/java/com/google/gson/DefaultDateTypeAdapterTest.java | 133 | gson/src/test/java/com/google/gson/DefaultDateTypeAdapterTest.java | testDateDeserializationISO8601 | /*
* Copyright (C) 2008 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.gson;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.Locale;
import java.util.TimeZone;
import junit.framework.TestCase;
/**
* A simple unit test for the {@link DefaultDateTypeAdapter} class.
*
* @author Joel Leitch
*/
public class DefaultDateTypeAdapterTest extends TestCase {
public void testFormattingInEnUs() {
assertFormattingAlwaysEmitsUsLocale(Locale.US);
}
public void testFormattingInFr() {
assertFormattingAlwaysEmitsUsLocale(Locale.FRANCE);
}
private void assertFormattingAlwaysEmitsUsLocale(Locale locale) {
TimeZone defaultTimeZone = TimeZone.getDefault();
TimeZone.setDefault(TimeZone.getTimeZone("UTC"));
Locale defaultLocale = Locale.getDefault();
Locale.setDefault(locale);
try {
assertFormatted("Jan 1, 1970 12:00:00 AM", new DefaultDateTypeAdapter());
assertFormatted("1/1/70", new DefaultDateTypeAdapter(DateFormat.SHORT));
assertFormatted("Jan 1, 1970", new DefaultDateTypeAdapter(DateFormat.MEDIUM));
assertFormatted("January 1, 1970", new DefaultDateTypeAdapter(DateFormat.LONG));
assertFormatted("1/1/70 12:00 AM",
new DefaultDateTypeAdapter(DateFormat.SHORT, DateFormat.SHORT));
assertFormatted("Jan 1, 1970 12:00:00 AM",
new DefaultDateTypeAdapter(DateFormat.MEDIUM, DateFormat.MEDIUM));
assertFormatted("January 1, 1970 12:00:00 AM UTC",
new DefaultDateTypeAdapter(DateFormat.LONG, DateFormat.LONG));
assertFormatted("Thursday, January 1, 1970 12:00:00 AM UTC",
new DefaultDateTypeAdapter(DateFormat.FULL, DateFormat.FULL));
} finally {
TimeZone.setDefault(defaultTimeZone);
Locale.setDefault(defaultLocale);
}
}
public void testParsingDatesFormattedWithSystemLocale() {
TimeZone defaultTimeZone = TimeZone.getDefault();
TimeZone.setDefault(TimeZone.getTimeZone("UTC"));
Locale defaultLocale = Locale.getDefault();
Locale.setDefault(Locale.FRANCE);
try {
assertParsed("1 janv. 1970 00:00:00", new DefaultDateTypeAdapter());
assertParsed("01/01/70", new DefaultDateTypeAdapter(DateFormat.SHORT));
assertParsed("1 janv. 1970", new DefaultDateTypeAdapter(DateFormat.MEDIUM));
assertParsed("1 janvier 1970", new DefaultDateTypeAdapter(DateFormat.LONG));
assertParsed("01/01/70 00:00",
new DefaultDateTypeAdapter(DateFormat.SHORT, DateFormat.SHORT));
assertParsed("1 janv. 1970 00:00:00",
new DefaultDateTypeAdapter(DateFormat.MEDIUM, DateFormat.MEDIUM));
assertParsed("1 janvier 1970 00:00:00 UTC",
new DefaultDateTypeAdapter(DateFormat.LONG, DateFormat.LONG));
assertParsed("jeudi 1 janvier 1970 00 h 00 UTC",
new DefaultDateTypeAdapter(DateFormat.FULL, DateFormat.FULL));
} finally {
TimeZone.setDefault(defaultTimeZone);
Locale.setDefault(defaultLocale);
}
}
public void testParsingDatesFormattedWithUsLocale() {
TimeZone defaultTimeZone = TimeZone.getDefault();
TimeZone.setDefault(TimeZone.getTimeZone("UTC"));
Locale defaultLocale = Locale.getDefault();
Locale.setDefault(Locale.US);
try {
assertParsed("Jan 1, 1970 0:00:00 AM", new DefaultDateTypeAdapter());
assertParsed("1/1/70", new DefaultDateTypeAdapter(DateFormat.SHORT));
assertParsed("Jan 1, 1970", new DefaultDateTypeAdapter(DateFormat.MEDIUM));
assertParsed("January 1, 1970", new DefaultDateTypeAdapter(DateFormat.LONG));
assertParsed("1/1/70 0:00 AM",
new DefaultDateTypeAdapter(DateFormat.SHORT, DateFormat.SHORT));
assertParsed("Jan 1, 1970 0:00:00 AM",
new DefaultDateTypeAdapter(DateFormat.MEDIUM, DateFormat.MEDIUM));
assertParsed("January 1, 1970 0:00:00 AM UTC",
new DefaultDateTypeAdapter(DateFormat.LONG, DateFormat.LONG));
assertParsed("Thursday, January 1, 1970 0:00:00 AM UTC",
new DefaultDateTypeAdapter(DateFormat.FULL, DateFormat.FULL));
} finally {
TimeZone.setDefault(defaultTimeZone);
Locale.setDefault(defaultLocale);
}
}
public void testFormatUsesDefaultTimezone() {
TimeZone defaultTimeZone = TimeZone.getDefault();
TimeZone.setDefault(TimeZone.getTimeZone("America/Los_Angeles"));
Locale defaultLocale = Locale.getDefault();
Locale.setDefault(Locale.US);
try {
assertFormatted("Dec 31, 1969 4:00:00 PM", new DefaultDateTypeAdapter());
assertParsed("Dec 31, 1969 4:00:00 PM", new DefaultDateTypeAdapter());
} finally {
TimeZone.setDefault(defaultTimeZone);
Locale.setDefault(defaultLocale);
}
}
public void testDateDeserializationISO8601() throws Exception {
DefaultDateTypeAdapter adapter = new DefaultDateTypeAdapter();
assertParsed("1970-01-01T00:00:00.000Z", adapter);
assertParsed("1970-01-01T00:00Z", adapter);
assertParsed("1970-01-01T00:00:00+00:00", adapter);
assertParsed("1970-01-01T01:00:00+01:00", adapter);
assertParsed("1970-01-01T01:00:00+01", adapter);
}
public void testDateSerialization() throws Exception {
int dateStyle = DateFormat.LONG;
DefaultDateTypeAdapter dateTypeAdapter = new DefaultDateTypeAdapter(dateStyle);
DateFormat formatter = DateFormat.getDateInstance(dateStyle, Locale.US);
Date currentDate = new Date();
String dateString = dateTypeAdapter.serialize(currentDate, Date.class, null).getAsString();
assertEquals(formatter.format(currentDate), dateString);
}
public void testDatePattern() throws Exception {
String pattern = "yyyy-MM-dd";
DefaultDateTypeAdapter dateTypeAdapter = new DefaultDateTypeAdapter(pattern);
DateFormat formatter = new SimpleDateFormat(pattern);
Date currentDate = new Date();
String dateString = dateTypeAdapter.serialize(currentDate, Date.class, null).getAsString();
assertEquals(formatter.format(currentDate), dateString);
}
public void testInvalidDatePattern() throws Exception {
try {
new DefaultDateTypeAdapter("I am a bad Date pattern....");
fail("Invalid date pattern should fail.");
} catch (IllegalArgumentException expected) { }
}
private void assertFormatted(String formatted, DefaultDateTypeAdapter adapter) {
assertEquals(formatted, adapter.serialize(new Date(0), Date.class, null).getAsString());
}
private void assertParsed(String date, DefaultDateTypeAdapter adapter) {
assertEquals(date, new Date(0), adapter.deserialize(new JsonPrimitive(date), Date.class, null));
assertEquals("ISO 8601", new Date(0), adapter.deserialize(
new JsonPrimitive("1970-01-01T00:00:00Z"), Date.class, null));
}
} | // You are a professional Java test case writer, please create a test case named `testDateDeserializationISO8601` for the issue `Gson-768`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Gson-768
//
// ## Issue-Title:
// ISO8601 is not fully implemented
//
// ## Issue-Description:
// Hi guys,
//
//
// I'm working on a project where I have to parse `2016-01-11T11:06:14.000-02` to java.util.Date which is a valid date according to [RFC3339](https://www.ietf.org/rfc/rfc3339.txt) on page 12.
//
//
// But I got an Exception trying to archive it
//
//
//
// ```
// Caused by: com.google.gson.JsonSyntaxException: 2016-01-11T11:06:14.000-02
// at com.google.gson.DefaultDateTypeAdapter.deserializeToDate(DefaultDateTypeAdapter.java:107)
// at com.google.gson.DefaultDateTypeAdapter.deserialize(DefaultDateTypeAdapter.java:84)
// at com.google.gson.DefaultDateTypeAdapter.deserialize(DefaultDateTypeAdapter.java:38)
// at com.google.gson.TreeTypeAdapter.read(TreeTypeAdapter.java:58)
// at com.google.gson.internal.bind.ReflectiveTypeAdapterFactory$1.read(ReflectiveTypeAdapterFactory.java:117)
// at com.google.gson.internal.bind.ReflectiveTypeAdapterFactory$Adapter.read(ReflectiveTypeAdapterFactory.java:217)
// at com.google.gson.internal.bind.TypeAdapterRuntimeTypeWrapper.read(TypeAdapterRuntimeTypeWrapper.java:40)
// at com.google.gson.internal.bind.CollectionTypeAdapterFactory$Adapter.read(CollectionTypeAdapterFactory.java:82)
// at com.google.gson.internal.bind.CollectionTypeAdapterFactory$Adapter.read(CollectionTypeAdapterFactory.java:61)
// at com.google.gson.internal.bind.ReflectiveTypeAdapterFactory$1.read(ReflectiveTypeAdapterFactory.java:117)
// at com.google.gson.internal.bind.ReflectiveTypeAdapterFactory$Adapter.read(ReflectiveTypeAdapterFactory.java:217)
// at com.google.gson.Gson.fromJson(Gson.java:861)
// at com.google.gson.Gson.fromJson(Gson.java:926)
// at com.google.gson.Gson.fromJson(Gson.java:899)
// at ...
// Caused by: java.text.ParseException: Failed to parse date ["2016-01-11T11:06:14.000-02']: Mismatching time zone indicator: GMT-02 given, resolves to GMT-02:00
// at com.google.gson.internal.bind.util.ISO8601Utils.parse(ISO8601Utils.java:270)
// at com.google.gson.DefaultDateTypeAdapter.deserializeToDate(DefaultDateTypeAdapter.java:105)
// ... 31 more
// Caused by: java.lang.IndexOutOfBoundsException: Mismatching time zone indicator: GMT-02 given, resolves to GMT-02:00
// at com.google.gson.internal.bind.util.ISO8601Utils.parse(ISO8601Utils.java:236)
// ... 32 more
//
// ```
//
// I'm able to fix this if it sounds reasonable.
//
//
//
//
public void testDateDeserializationISO8601() throws Exception {
| 133 | 5 | 126 | gson/src/test/java/com/google/gson/DefaultDateTypeAdapterTest.java | gson/src/test/java | ```markdown
## Issue-ID: Gson-768
## Issue-Title:
ISO8601 is not fully implemented
## Issue-Description:
Hi guys,
I'm working on a project where I have to parse `2016-01-11T11:06:14.000-02` to java.util.Date which is a valid date according to [RFC3339](https://www.ietf.org/rfc/rfc3339.txt) on page 12.
But I got an Exception trying to archive it
```
Caused by: com.google.gson.JsonSyntaxException: 2016-01-11T11:06:14.000-02
at com.google.gson.DefaultDateTypeAdapter.deserializeToDate(DefaultDateTypeAdapter.java:107)
at com.google.gson.DefaultDateTypeAdapter.deserialize(DefaultDateTypeAdapter.java:84)
at com.google.gson.DefaultDateTypeAdapter.deserialize(DefaultDateTypeAdapter.java:38)
at com.google.gson.TreeTypeAdapter.read(TreeTypeAdapter.java:58)
at com.google.gson.internal.bind.ReflectiveTypeAdapterFactory$1.read(ReflectiveTypeAdapterFactory.java:117)
at com.google.gson.internal.bind.ReflectiveTypeAdapterFactory$Adapter.read(ReflectiveTypeAdapterFactory.java:217)
at com.google.gson.internal.bind.TypeAdapterRuntimeTypeWrapper.read(TypeAdapterRuntimeTypeWrapper.java:40)
at com.google.gson.internal.bind.CollectionTypeAdapterFactory$Adapter.read(CollectionTypeAdapterFactory.java:82)
at com.google.gson.internal.bind.CollectionTypeAdapterFactory$Adapter.read(CollectionTypeAdapterFactory.java:61)
at com.google.gson.internal.bind.ReflectiveTypeAdapterFactory$1.read(ReflectiveTypeAdapterFactory.java:117)
at com.google.gson.internal.bind.ReflectiveTypeAdapterFactory$Adapter.read(ReflectiveTypeAdapterFactory.java:217)
at com.google.gson.Gson.fromJson(Gson.java:861)
at com.google.gson.Gson.fromJson(Gson.java:926)
at com.google.gson.Gson.fromJson(Gson.java:899)
at ...
Caused by: java.text.ParseException: Failed to parse date ["2016-01-11T11:06:14.000-02']: Mismatching time zone indicator: GMT-02 given, resolves to GMT-02:00
at com.google.gson.internal.bind.util.ISO8601Utils.parse(ISO8601Utils.java:270)
at com.google.gson.DefaultDateTypeAdapter.deserializeToDate(DefaultDateTypeAdapter.java:105)
... 31 more
Caused by: java.lang.IndexOutOfBoundsException: Mismatching time zone indicator: GMT-02 given, resolves to GMT-02:00
at com.google.gson.internal.bind.util.ISO8601Utils.parse(ISO8601Utils.java:236)
... 32 more
```
I'm able to fix this if it sounds reasonable.
```
You are a professional Java test case writer, please create a test case named `testDateDeserializationISO8601` for the issue `Gson-768`, utilizing the provided issue report information and the following function signature.
```java
public void testDateDeserializationISO8601() throws Exception {
```
| 126 | [
"com.google.gson.internal.bind.util.ISO8601Utils"
] | 733aff847b35cde65be97e0607dba0d99d2584c7e558e50683de67fd60127c34 | public void testDateDeserializationISO8601() throws Exception | // You are a professional Java test case writer, please create a test case named `testDateDeserializationISO8601` for the issue `Gson-768`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Gson-768
//
// ## Issue-Title:
// ISO8601 is not fully implemented
//
// ## Issue-Description:
// Hi guys,
//
//
// I'm working on a project where I have to parse `2016-01-11T11:06:14.000-02` to java.util.Date which is a valid date according to [RFC3339](https://www.ietf.org/rfc/rfc3339.txt) on page 12.
//
//
// But I got an Exception trying to archive it
//
//
//
// ```
// Caused by: com.google.gson.JsonSyntaxException: 2016-01-11T11:06:14.000-02
// at com.google.gson.DefaultDateTypeAdapter.deserializeToDate(DefaultDateTypeAdapter.java:107)
// at com.google.gson.DefaultDateTypeAdapter.deserialize(DefaultDateTypeAdapter.java:84)
// at com.google.gson.DefaultDateTypeAdapter.deserialize(DefaultDateTypeAdapter.java:38)
// at com.google.gson.TreeTypeAdapter.read(TreeTypeAdapter.java:58)
// at com.google.gson.internal.bind.ReflectiveTypeAdapterFactory$1.read(ReflectiveTypeAdapterFactory.java:117)
// at com.google.gson.internal.bind.ReflectiveTypeAdapterFactory$Adapter.read(ReflectiveTypeAdapterFactory.java:217)
// at com.google.gson.internal.bind.TypeAdapterRuntimeTypeWrapper.read(TypeAdapterRuntimeTypeWrapper.java:40)
// at com.google.gson.internal.bind.CollectionTypeAdapterFactory$Adapter.read(CollectionTypeAdapterFactory.java:82)
// at com.google.gson.internal.bind.CollectionTypeAdapterFactory$Adapter.read(CollectionTypeAdapterFactory.java:61)
// at com.google.gson.internal.bind.ReflectiveTypeAdapterFactory$1.read(ReflectiveTypeAdapterFactory.java:117)
// at com.google.gson.internal.bind.ReflectiveTypeAdapterFactory$Adapter.read(ReflectiveTypeAdapterFactory.java:217)
// at com.google.gson.Gson.fromJson(Gson.java:861)
// at com.google.gson.Gson.fromJson(Gson.java:926)
// at com.google.gson.Gson.fromJson(Gson.java:899)
// at ...
// Caused by: java.text.ParseException: Failed to parse date ["2016-01-11T11:06:14.000-02']: Mismatching time zone indicator: GMT-02 given, resolves to GMT-02:00
// at com.google.gson.internal.bind.util.ISO8601Utils.parse(ISO8601Utils.java:270)
// at com.google.gson.DefaultDateTypeAdapter.deserializeToDate(DefaultDateTypeAdapter.java:105)
// ... 31 more
// Caused by: java.lang.IndexOutOfBoundsException: Mismatching time zone indicator: GMT-02 given, resolves to GMT-02:00
// at com.google.gson.internal.bind.util.ISO8601Utils.parse(ISO8601Utils.java:236)
// ... 32 more
//
// ```
//
// I'm able to fix this if it sounds reasonable.
//
//
//
//
| Gson | /*
* Copyright (C) 2008 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.gson;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.Locale;
import java.util.TimeZone;
import junit.framework.TestCase;
/**
* A simple unit test for the {@link DefaultDateTypeAdapter} class.
*
* @author Joel Leitch
*/
public class DefaultDateTypeAdapterTest extends TestCase {
public void testFormattingInEnUs() {
assertFormattingAlwaysEmitsUsLocale(Locale.US);
}
public void testFormattingInFr() {
assertFormattingAlwaysEmitsUsLocale(Locale.FRANCE);
}
private void assertFormattingAlwaysEmitsUsLocale(Locale locale) {
TimeZone defaultTimeZone = TimeZone.getDefault();
TimeZone.setDefault(TimeZone.getTimeZone("UTC"));
Locale defaultLocale = Locale.getDefault();
Locale.setDefault(locale);
try {
assertFormatted("Jan 1, 1970 12:00:00 AM", new DefaultDateTypeAdapter());
assertFormatted("1/1/70", new DefaultDateTypeAdapter(DateFormat.SHORT));
assertFormatted("Jan 1, 1970", new DefaultDateTypeAdapter(DateFormat.MEDIUM));
assertFormatted("January 1, 1970", new DefaultDateTypeAdapter(DateFormat.LONG));
assertFormatted("1/1/70 12:00 AM",
new DefaultDateTypeAdapter(DateFormat.SHORT, DateFormat.SHORT));
assertFormatted("Jan 1, 1970 12:00:00 AM",
new DefaultDateTypeAdapter(DateFormat.MEDIUM, DateFormat.MEDIUM));
assertFormatted("January 1, 1970 12:00:00 AM UTC",
new DefaultDateTypeAdapter(DateFormat.LONG, DateFormat.LONG));
assertFormatted("Thursday, January 1, 1970 12:00:00 AM UTC",
new DefaultDateTypeAdapter(DateFormat.FULL, DateFormat.FULL));
} finally {
TimeZone.setDefault(defaultTimeZone);
Locale.setDefault(defaultLocale);
}
}
public void testParsingDatesFormattedWithSystemLocale() {
TimeZone defaultTimeZone = TimeZone.getDefault();
TimeZone.setDefault(TimeZone.getTimeZone("UTC"));
Locale defaultLocale = Locale.getDefault();
Locale.setDefault(Locale.FRANCE);
try {
assertParsed("1 janv. 1970 00:00:00", new DefaultDateTypeAdapter());
assertParsed("01/01/70", new DefaultDateTypeAdapter(DateFormat.SHORT));
assertParsed("1 janv. 1970", new DefaultDateTypeAdapter(DateFormat.MEDIUM));
assertParsed("1 janvier 1970", new DefaultDateTypeAdapter(DateFormat.LONG));
assertParsed("01/01/70 00:00",
new DefaultDateTypeAdapter(DateFormat.SHORT, DateFormat.SHORT));
assertParsed("1 janv. 1970 00:00:00",
new DefaultDateTypeAdapter(DateFormat.MEDIUM, DateFormat.MEDIUM));
assertParsed("1 janvier 1970 00:00:00 UTC",
new DefaultDateTypeAdapter(DateFormat.LONG, DateFormat.LONG));
assertParsed("jeudi 1 janvier 1970 00 h 00 UTC",
new DefaultDateTypeAdapter(DateFormat.FULL, DateFormat.FULL));
} finally {
TimeZone.setDefault(defaultTimeZone);
Locale.setDefault(defaultLocale);
}
}
public void testParsingDatesFormattedWithUsLocale() {
TimeZone defaultTimeZone = TimeZone.getDefault();
TimeZone.setDefault(TimeZone.getTimeZone("UTC"));
Locale defaultLocale = Locale.getDefault();
Locale.setDefault(Locale.US);
try {
assertParsed("Jan 1, 1970 0:00:00 AM", new DefaultDateTypeAdapter());
assertParsed("1/1/70", new DefaultDateTypeAdapter(DateFormat.SHORT));
assertParsed("Jan 1, 1970", new DefaultDateTypeAdapter(DateFormat.MEDIUM));
assertParsed("January 1, 1970", new DefaultDateTypeAdapter(DateFormat.LONG));
assertParsed("1/1/70 0:00 AM",
new DefaultDateTypeAdapter(DateFormat.SHORT, DateFormat.SHORT));
assertParsed("Jan 1, 1970 0:00:00 AM",
new DefaultDateTypeAdapter(DateFormat.MEDIUM, DateFormat.MEDIUM));
assertParsed("January 1, 1970 0:00:00 AM UTC",
new DefaultDateTypeAdapter(DateFormat.LONG, DateFormat.LONG));
assertParsed("Thursday, January 1, 1970 0:00:00 AM UTC",
new DefaultDateTypeAdapter(DateFormat.FULL, DateFormat.FULL));
} finally {
TimeZone.setDefault(defaultTimeZone);
Locale.setDefault(defaultLocale);
}
}
public void testFormatUsesDefaultTimezone() {
TimeZone defaultTimeZone = TimeZone.getDefault();
TimeZone.setDefault(TimeZone.getTimeZone("America/Los_Angeles"));
Locale defaultLocale = Locale.getDefault();
Locale.setDefault(Locale.US);
try {
assertFormatted("Dec 31, 1969 4:00:00 PM", new DefaultDateTypeAdapter());
assertParsed("Dec 31, 1969 4:00:00 PM", new DefaultDateTypeAdapter());
} finally {
TimeZone.setDefault(defaultTimeZone);
Locale.setDefault(defaultLocale);
}
}
public void testDateDeserializationISO8601() throws Exception {
DefaultDateTypeAdapter adapter = new DefaultDateTypeAdapter();
assertParsed("1970-01-01T00:00:00.000Z", adapter);
assertParsed("1970-01-01T00:00Z", adapter);
assertParsed("1970-01-01T00:00:00+00:00", adapter);
assertParsed("1970-01-01T01:00:00+01:00", adapter);
assertParsed("1970-01-01T01:00:00+01", adapter);
}
public void testDateSerialization() throws Exception {
int dateStyle = DateFormat.LONG;
DefaultDateTypeAdapter dateTypeAdapter = new DefaultDateTypeAdapter(dateStyle);
DateFormat formatter = DateFormat.getDateInstance(dateStyle, Locale.US);
Date currentDate = new Date();
String dateString = dateTypeAdapter.serialize(currentDate, Date.class, null).getAsString();
assertEquals(formatter.format(currentDate), dateString);
}
public void testDatePattern() throws Exception {
String pattern = "yyyy-MM-dd";
DefaultDateTypeAdapter dateTypeAdapter = new DefaultDateTypeAdapter(pattern);
DateFormat formatter = new SimpleDateFormat(pattern);
Date currentDate = new Date();
String dateString = dateTypeAdapter.serialize(currentDate, Date.class, null).getAsString();
assertEquals(formatter.format(currentDate), dateString);
}
public void testInvalidDatePattern() throws Exception {
try {
new DefaultDateTypeAdapter("I am a bad Date pattern....");
fail("Invalid date pattern should fail.");
} catch (IllegalArgumentException expected) { }
}
private void assertFormatted(String formatted, DefaultDateTypeAdapter adapter) {
assertEquals(formatted, adapter.serialize(new Date(0), Date.class, null).getAsString());
}
private void assertParsed(String date, DefaultDateTypeAdapter adapter) {
assertEquals(date, new Date(0), adapter.deserialize(new JsonPrimitive(date), Date.class, null));
assertEquals("ISO 8601", new Date(0), adapter.deserialize(
new JsonPrimitive("1970-01-01T00:00:00Z"), Date.class, null));
}
} |
||
@Test(expected=NumberIsTooLargeException.class)
public void testMath679() {
new OpenMapRealMatrix(3, Integer.MAX_VALUE);
} | org.apache.commons.math.linear.OpenMapRealMatrixTest::testMath679 | src/test/java/org/apache/commons/math/linear/OpenMapRealMatrixTest.java | 26 | src/test/java/org/apache/commons/math/linear/OpenMapRealMatrixTest.java | testMath679 | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.math.linear;
import org.apache.commons.math.exception.NumberIsTooLargeException;
import org.junit.Test;
public final class OpenMapRealMatrixTest {
@Test(expected=NumberIsTooLargeException.class)
public void testMath679() {
new OpenMapRealMatrix(3, Integer.MAX_VALUE);
}
} | // You are a professional Java test case writer, please create a test case named `testMath679` for the issue `Math-MATH-679`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Math-MATH-679
//
// ## Issue-Title:
// Integer overflow in OpenMapRealMatrix
//
// ## Issue-Description:
//
// computeKey() has an integer overflow. Since it is a sparse matrix, this is quite easily encountered long before heap space is exhausted. The attached code demonstrates the problem, which could potentially be a security vulnerability (for example, if one was to use this matrix to store access control information).
//
//
// Workaround: never create an OpenMapRealMatrix with more cells than are addressable with an int.
//
//
//
//
//
@Test(expected=NumberIsTooLargeException.class)
public void testMath679() {
| 26 | 45 | 23 | src/test/java/org/apache/commons/math/linear/OpenMapRealMatrixTest.java | src/test/java | ```markdown
## Issue-ID: Math-MATH-679
## Issue-Title:
Integer overflow in OpenMapRealMatrix
## Issue-Description:
computeKey() has an integer overflow. Since it is a sparse matrix, this is quite easily encountered long before heap space is exhausted. The attached code demonstrates the problem, which could potentially be a security vulnerability (for example, if one was to use this matrix to store access control information).
Workaround: never create an OpenMapRealMatrix with more cells than are addressable with an int.
```
You are a professional Java test case writer, please create a test case named `testMath679` for the issue `Math-MATH-679`, utilizing the provided issue report information and the following function signature.
```java
@Test(expected=NumberIsTooLargeException.class)
public void testMath679() {
```
| 23 | [
"org.apache.commons.math.linear.OpenMapRealMatrix"
] | 73bc8736369edf4e77025d9915a04473b0a08fbf311ba32ccae514351a3babc9 | @Test(expected=NumberIsTooLargeException.class)
public void testMath679() | // You are a professional Java test case writer, please create a test case named `testMath679` for the issue `Math-MATH-679`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Math-MATH-679
//
// ## Issue-Title:
// Integer overflow in OpenMapRealMatrix
//
// ## Issue-Description:
//
// computeKey() has an integer overflow. Since it is a sparse matrix, this is quite easily encountered long before heap space is exhausted. The attached code demonstrates the problem, which could potentially be a security vulnerability (for example, if one was to use this matrix to store access control information).
//
//
// Workaround: never create an OpenMapRealMatrix with more cells than are addressable with an int.
//
//
//
//
//
| Math | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.math.linear;
import org.apache.commons.math.exception.NumberIsTooLargeException;
import org.junit.Test;
public final class OpenMapRealMatrixTest {
@Test(expected=NumberIsTooLargeException.class)
public void testMath679() {
new OpenMapRealMatrix(3, Integer.MAX_VALUE);
}
} |
||
@Test
public void testGetLineNumberWithCR() throws Exception {
CSVParser parser = new CSVParser("a\rb\rc", CSVFormat.DEFAULT.withLineSeparator("\r"));
assertEquals(0, parser.getLineNumber());
assertNotNull(parser.getRecord());
assertEquals(1, parser.getLineNumber());
assertNotNull(parser.getRecord());
assertEquals(2, parser.getLineNumber());
assertNotNull(parser.getRecord());
assertEquals(2, parser.getLineNumber());
assertNull(parser.getRecord());
} | org.apache.commons.csv.CSVParserTest::testGetLineNumberWithCR | src/test/java/org/apache/commons/csv/CSVParserTest.java | 515 | src/test/java/org/apache/commons/csv/CSVParserTest.java | testGetLineNumberWithCR | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.csv;
import java.io.IOException;
import java.io.Reader;
import java.io.StringReader;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.NoSuchElementException;
import org.junit.Ignore;
import org.junit.Test;
import static org.junit.Assert.*;
/**
* CSVParserTest
*
* The test are organized in three different sections:
* The 'setter/getter' section, the lexer section and finally the parser
* section. In case a test fails, you should follow a top-down approach for
* fixing a potential bug (its likely that the parser itself fails if the lexer
* has problems...).
*/
public class CSVParserTest {
String code = "a,b,c,d\n"
+ " a , b , 1 2 \n"
+ "\"foo baar\", b,\n"
// + " \"foo\n,,\n\"\",,\n\\\"\",d,e\n";
+ " \"foo\n,,\n\"\",,\n\"\"\",d,e\n"; // changed to use standard CSV escaping
String[][] res = {
{"a", "b", "c", "d"},
{"a", "b", "1 2"},
{"foo baar", "b", ""},
{"foo\n,,\n\",,\n\"", "d", "e"}
};
@Test
public void testGetLine() throws IOException {
CSVParser parser = new CSVParser(new StringReader(code), CSVFormat.DEFAULT.withSurroundingSpacesIgnored(true));
for (String[] re : res) {
assertArrayEquals(re, parser.getRecord().values());
}
assertNull(parser.getRecord());
}
@Test
public void testGetRecords() throws IOException {
CSVParser parser = new CSVParser(new StringReader(code), CSVFormat.DEFAULT.withSurroundingSpacesIgnored(true));
List<CSVRecord> records = parser.getRecords();
assertEquals(res.length, records.size());
assertTrue(records.size() > 0);
for (int i = 0; i < res.length; i++) {
assertArrayEquals(res[i], records.get(i).values());
}
}
@Test
public void testExcelFormat1() throws IOException {
String code =
"value1,value2,value3,value4\r\na,b,c,d\r\n x,,,"
+ "\r\n\r\n\"\"\"hello\"\"\",\" \"\"world\"\"\",\"abc\ndef\",\r\n";
String[][] res = {
{"value1", "value2", "value3", "value4"},
{"a", "b", "c", "d"},
{" x", "", "", ""},
{""},
{"\"hello\"", " \"world\"", "abc\ndef", ""}
};
CSVParser parser = new CSVParser(code, CSVFormat.EXCEL);
List<CSVRecord> records = parser.getRecords();
assertEquals(res.length, records.size());
assertTrue(records.size() > 0);
for (int i = 0; i < res.length; i++) {
assertArrayEquals(res[i], records.get(i).values());
}
}
@Test
public void testExcelFormat2() throws Exception {
String code = "foo,baar\r\n\r\nhello,\r\n\r\nworld,\r\n";
String[][] res = {
{"foo", "baar"},
{""},
{"hello", ""},
{""},
{"world", ""}
};
CSVParser parser = new CSVParser(code, CSVFormat.EXCEL);
List<CSVRecord> records = parser.getRecords();
assertEquals(res.length, records.size());
assertTrue(records.size() > 0);
for (int i = 0; i < res.length; i++) {
assertArrayEquals(res[i], records.get(i).values());
}
}
@Test
public void testEndOfFileBehaviourExcel() throws Exception {
String[] codes = {
"hello,\r\n\r\nworld,\r\n",
"hello,\r\n\r\nworld,",
"hello,\r\n\r\nworld,\"\"\r\n",
"hello,\r\n\r\nworld,\"\"",
"hello,\r\n\r\nworld,\n",
"hello,\r\n\r\nworld,",
"hello,\r\n\r\nworld,\"\"\n",
"hello,\r\n\r\nworld,\"\""
};
String[][] res = {
{"hello", ""},
{""}, // Excel format does not ignore empty lines
{"world", ""}
};
for (String code : codes) {
CSVParser parser = new CSVParser(code, CSVFormat.EXCEL);
List<CSVRecord> records = parser.getRecords();
assertEquals(res.length, records.size());
assertTrue(records.size() > 0);
for (int i = 0; i < res.length; i++) {
assertArrayEquals(res[i], records.get(i).values());
}
}
}
@Test
public void testEndOfFileBehaviorCSV() throws Exception {
String[] codes = {
"hello,\r\n\r\nworld,\r\n",
"hello,\r\n\r\nworld,",
"hello,\r\n\r\nworld,\"\"\r\n",
"hello,\r\n\r\nworld,\"\"",
"hello,\r\n\r\nworld,\n",
"hello,\r\n\r\nworld,",
"hello,\r\n\r\nworld,\"\"\n",
"hello,\r\n\r\nworld,\"\""
};
String[][] res = {
{"hello", ""}, // CSV format ignores empty lines
{"world", ""}
};
for (String code : codes) {
CSVParser parser = new CSVParser(new StringReader(code));
List<CSVRecord> records = parser.getRecords();
assertEquals(res.length, records.size());
assertTrue(records.size() > 0);
for (int i = 0; i < res.length; i++) {
assertArrayEquals(res[i], records.get(i).values());
}
}
}
@Test
public void testEmptyLineBehaviourExcel() throws Exception {
String[] codes = {
"hello,\r\n\r\n\r\n",
"hello,\n\n\n",
"hello,\"\"\r\n\r\n\r\n",
"hello,\"\"\n\n\n"
};
String[][] res = {
{"hello", ""},
{""}, // Excel format does not ignore empty lines
{""}
};
for (String code : codes) {
CSVParser parser = new CSVParser(code, CSVFormat.EXCEL);
List<CSVRecord> records = parser.getRecords();
assertEquals(res.length, records.size());
assertTrue(records.size() > 0);
for (int i = 0; i < res.length; i++) {
assertArrayEquals(res[i], records.get(i).values());
}
}
}
@Test
public void testEmptyLineBehaviourCSV() throws Exception {
String[] codes = {
"hello,\r\n\r\n\r\n",
"hello,\n\n\n",
"hello,\"\"\r\n\r\n\r\n",
"hello,\"\"\n\n\n"
};
String[][] res = {
{"hello", ""} // CSV format ignores empty lines
};
for (String code : codes) {
CSVParser parser = new CSVParser(new StringReader(code));
List<CSVRecord> records = parser.getRecords();
assertEquals(res.length, records.size());
assertTrue(records.size() > 0);
for (int i = 0; i < res.length; i++) {
assertArrayEquals(res[i], records.get(i).values());
}
}
}
@Test
public void testEmptyFile() throws Exception {
CSVParser parser = new CSVParser("", CSVFormat.DEFAULT);
assertNull(parser.getRecord());
}
@Test
@Ignore
public void testBackslashEscapingOld() throws IOException {
String code =
"one,two,three\n"
+ "on\\\"e,two\n"
+ "on\"e,two\n"
+ "one,\"tw\\\"o\"\n"
+ "one,\"t\\,wo\"\n"
+ "one,two,\"th,ree\"\n"
+ "\"a\\\\\"\n"
+ "a\\,b\n"
+ "\"a\\\\,b\"";
String[][] res = {
{"one", "two", "three"},
{"on\\\"e", "two"},
{"on\"e", "two"},
{"one", "tw\"o"},
{"one", "t\\,wo"}, // backslash in quotes only escapes a delimiter (",")
{"one", "two", "th,ree"},
{"a\\\\"}, // backslash in quotes only escapes a delimiter (",")
{"a\\", "b"}, // a backslash must be returnd
{"a\\\\,b"} // backslash in quotes only escapes a delimiter (",")
};
CSVParser parser = new CSVParser(new StringReader(code));
List<CSVRecord> records = parser.getRecords();
assertEquals(res.length, records.size());
assertTrue(records.size() > 0);
for (int i = 0; i < res.length; i++) {
assertArrayEquals(res[i], records.get(i).values());
}
}
@Test
public void testBackslashEscaping() throws IOException {
// To avoid confusion over the need for escaping chars in java code,
// We will test with a forward slash as the escape char, and a single
// quote as the encapsulator.
String code =
"one,two,three\n" // 0
+ "'',''\n" // 1) empty encapsulators
+ "/',/'\n" // 2) single encapsulators
+ "'/'','/''\n" // 3) single encapsulators encapsulated via escape
+ "'''',''''\n" // 4) single encapsulators encapsulated via doubling
+ "/,,/,\n" // 5) separator escaped
+ "//,//\n" // 6) escape escaped
+ "'//','//'\n" // 7) escape escaped in encapsulation
+ " 8 , \"quoted \"\" /\" // string\" \n" // don't eat spaces
+ "9, /\n \n" // escaped newline
+ "";
String[][] res = {
{"one", "two", "three"}, // 0
{"", ""}, // 1
{"'", "'"}, // 2
{"'", "'"}, // 3
{"'", "'"}, // 4
{",", ","}, // 5
{"/", "/"}, // 6
{"/", "/"}, // 7
{" 8 ", " \"quoted \"\" \" / string\" "},
{"9", " \n "},
};
CSVFormat format = new CSVFormat(',', '\'', CSVFormat.DISABLED, '/', false, true, "\r\n", null);
CSVParser parser = new CSVParser(code, format);
List<CSVRecord> records = parser.getRecords();
assertTrue(records.size() > 0);
for (int i = 0; i < res.length; i++) {
assertArrayEquals(res[i], records.get(i).values());
}
}
@Test
public void testBackslashEscaping2() throws IOException {
// To avoid confusion over the need for escaping chars in java code,
// We will test with a forward slash as the escape char, and a single
// quote as the encapsulator.
String code = ""
+ " , , \n" // 1)
+ " \t , , \n" // 2)
+ " // , /, , /,\n" // 3)
+ "";
String[][] res = {
{" ", " ", " "}, // 1
{" \t ", " ", " "}, // 2
{" / ", " , ", " ,"}, // 3
};
CSVFormat format = new CSVFormat(',', CSVFormat.DISABLED, CSVFormat.DISABLED, '/', false, true, "\r\n", null);
CSVParser parser = new CSVParser(code, format);
List<CSVRecord> records = parser.getRecords();
assertTrue(records.size() > 0);
assertTrue(CSVPrinterTest.equals(res, records));
}
@Test
public void testDefaultFormat() throws IOException {
String code = ""
+ "a,b\n" // 1)
+ "\"\n\",\" \"\n" // 2)
+ "\"\",#\n" // 2)
;
String[][] res = {
{"a", "b"},
{"\n", " "},
{"", "#"},
};
CSVFormat format = CSVFormat.DEFAULT;
assertEquals(CSVFormat.DISABLED, format.getCommentStart());
CSVParser parser = new CSVParser(code, format);
List<CSVRecord> records = parser.getRecords();
assertTrue(records.size() > 0);
assertTrue(CSVPrinterTest.equals(res, records));
String[][] res_comments = {
{"a", "b"},
{"\n", " "},
{""},
};
format = CSVFormat.DEFAULT.withCommentStart('#');
parser = new CSVParser(code, format);
records = parser.getRecords();
assertTrue(CSVPrinterTest.equals(res_comments, records));
}
@Test
public void testCarriageReturnLineFeedEndings() throws IOException {
String code = "foo\r\nbaar,\r\nhello,world\r\n,kanu";
CSVParser parser = new CSVParser(new StringReader(code));
List<CSVRecord> records = parser.getRecords();
assertEquals(4, records.size());
}
@Test
public void testCarriageReturnEndings() throws IOException {
String code = "foo\rbaar,\rhello,world\r,kanu";
CSVParser parser = new CSVParser(new StringReader(code));
List<CSVRecord> records = parser.getRecords();
assertEquals(4, records.size());
}
@Test
public void testLineFeedEndings() throws IOException {
String code = "foo\nbaar,\nhello,world\n,kanu";
CSVParser parser = new CSVParser(new StringReader(code));
List<CSVRecord> records = parser.getRecords();
assertEquals(4, records.size());
}
@Test
public void testIgnoreEmptyLines() throws IOException {
String code = "\nfoo,baar\n\r\n,\n\n,world\r\n\n";
//String code = "world\r\n\n";
//String code = "foo;baar\r\n\r\nhello;\r\n\r\nworld;\r\n";
CSVParser parser = new CSVParser(new StringReader(code));
List<CSVRecord> records = parser.getRecords();
assertEquals(3, records.size());
}
@Test
public void testForEach() throws Exception {
List<CSVRecord> records = new ArrayList<CSVRecord>();
Reader in = new StringReader("a,b,c\n1,2,3\nx,y,z");
for (CSVRecord record : CSVFormat.DEFAULT.parse(in)) {
records.add(record);
}
assertEquals(3, records.size());
assertArrayEquals(new String[]{"a", "b", "c"}, records.get(0).values());
assertArrayEquals(new String[]{"1", "2", "3"}, records.get(1).values());
assertArrayEquals(new String[]{"x", "y", "z"}, records.get(2).values());
}
@Test
public void testIterator() throws Exception {
Reader in = new StringReader("a,b,c\n1,2,3\nx,y,z");
Iterator<CSVRecord> iterator = CSVFormat.DEFAULT.parse(in).iterator();
assertTrue(iterator.hasNext());
try {
iterator.remove();
fail("expected UnsupportedOperationException");
} catch (UnsupportedOperationException expected) {
}
assertArrayEquals(new String[]{"a", "b", "c"}, iterator.next().values());
assertArrayEquals(new String[]{"1", "2", "3"}, iterator.next().values());
assertTrue(iterator.hasNext());
assertTrue(iterator.hasNext());
assertTrue(iterator.hasNext());
assertArrayEquals(new String[]{"x", "y", "z"}, iterator.next().values());
assertFalse(iterator.hasNext());
try {
iterator.next();
fail("NoSuchElementException expected");
} catch (NoSuchElementException e) {
// expected
}
}
@Test
public void testHeader() throws Exception {
Reader in = new StringReader("a,b,c\n1,2,3\nx,y,z");
Iterator<CSVRecord> records = CSVFormat.DEFAULT.withHeader().parse(in).iterator();
for (int i = 0; i < 2; i++) {
assertTrue(records.hasNext());
CSVRecord record = records.next();
assertEquals(record.get(0), record.get("a"));
assertEquals(record.get(1), record.get("b"));
assertEquals(record.get(2), record.get("c"));
}
assertFalse(records.hasNext());
}
@Test
public void testProvidedHeader() throws Exception {
Reader in = new StringReader("a,b,c\n1,2,3\nx,y,z");
Iterator<CSVRecord> records = CSVFormat.DEFAULT.withHeader("A", "B", "C").parse(in).iterator();
for (int i = 0; i < 3; i++) {
assertTrue(records.hasNext());
CSVRecord record = records.next();
assertEquals(record.get(0), record.get("A"));
assertEquals(record.get(1), record.get("B"));
assertEquals(record.get(2), record.get("C"));
}
assertFalse(records.hasNext());
}
@Test
public void testGetLineNumberWithLF() throws Exception {
CSVParser parser = new CSVParser("a\nb\nc", CSVFormat.DEFAULT.withLineSeparator("\n"));
assertEquals(0, parser.getLineNumber());
assertNotNull(parser.getRecord());
assertEquals(1, parser.getLineNumber());
assertNotNull(parser.getRecord());
assertEquals(2, parser.getLineNumber());
assertNotNull(parser.getRecord());
assertEquals(2, parser.getLineNumber());
assertNull(parser.getRecord());
}
@Test
public void testGetLineNumberWithCRLF() throws Exception {
CSVParser parser = new CSVParser("a\r\nb\r\nc", CSVFormat.DEFAULT.withLineSeparator("\r\n"));
assertEquals(0, parser.getLineNumber());
assertNotNull(parser.getRecord());
assertEquals(1, parser.getLineNumber());
assertNotNull(parser.getRecord());
assertEquals(2, parser.getLineNumber());
assertNotNull(parser.getRecord());
assertEquals(2, parser.getLineNumber());
assertNull(parser.getRecord());
}
@Test
public void testGetLineNumberWithCR() throws Exception {
CSVParser parser = new CSVParser("a\rb\rc", CSVFormat.DEFAULT.withLineSeparator("\r"));
assertEquals(0, parser.getLineNumber());
assertNotNull(parser.getRecord());
assertEquals(1, parser.getLineNumber());
assertNotNull(parser.getRecord());
assertEquals(2, parser.getLineNumber());
assertNotNull(parser.getRecord());
assertEquals(2, parser.getLineNumber());
assertNull(parser.getRecord());
}
} | // You are a professional Java test case writer, please create a test case named `testGetLineNumberWithCR` for the issue `Csv-CSV-75`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Csv-CSV-75
//
// ## Issue-Title:
// ExtendedBufferReader does not handle EOL consistently
//
// ## Issue-Description:
//
// ExtendedBufferReader checks for '\n' (LF) in the read() methods, incrementing linecount when found.
//
//
// However, the readLine() method calls BufferedReader.readLine() which treats CR, LF and CRLF equally (and drops them).
//
//
// If the code is to be flexible in what it accepts, the class should also allow for CR alone as a line terminator.
//
//
// It should work if the code increments the line counter for CR, and for LF if the previous character was not CR.
//
//
//
//
//
@Test
public void testGetLineNumberWithCR() throws Exception {
| 515 | 1 | 503 | src/test/java/org/apache/commons/csv/CSVParserTest.java | src/test/java | ```markdown
## Issue-ID: Csv-CSV-75
## Issue-Title:
ExtendedBufferReader does not handle EOL consistently
## Issue-Description:
ExtendedBufferReader checks for '\n' (LF) in the read() methods, incrementing linecount when found.
However, the readLine() method calls BufferedReader.readLine() which treats CR, LF and CRLF equally (and drops them).
If the code is to be flexible in what it accepts, the class should also allow for CR alone as a line terminator.
It should work if the code increments the line counter for CR, and for LF if the previous character was not CR.
```
You are a professional Java test case writer, please create a test case named `testGetLineNumberWithCR` for the issue `Csv-CSV-75`, utilizing the provided issue report information and the following function signature.
```java
@Test
public void testGetLineNumberWithCR() throws Exception {
```
| 503 | [
"org.apache.commons.csv.ExtendedBufferedReader"
] | 74479ffa5f3d1eff5086f90f54cc4990d312d974eb83e567fb0f734d971f2bfb | @Test
public void testGetLineNumberWithCR() throws Exception | // You are a professional Java test case writer, please create a test case named `testGetLineNumberWithCR` for the issue `Csv-CSV-75`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Csv-CSV-75
//
// ## Issue-Title:
// ExtendedBufferReader does not handle EOL consistently
//
// ## Issue-Description:
//
// ExtendedBufferReader checks for '\n' (LF) in the read() methods, incrementing linecount when found.
//
//
// However, the readLine() method calls BufferedReader.readLine() which treats CR, LF and CRLF equally (and drops them).
//
//
// If the code is to be flexible in what it accepts, the class should also allow for CR alone as a line terminator.
//
//
// It should work if the code increments the line counter for CR, and for LF if the previous character was not CR.
//
//
//
//
//
| Csv | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.csv;
import java.io.IOException;
import java.io.Reader;
import java.io.StringReader;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.NoSuchElementException;
import org.junit.Ignore;
import org.junit.Test;
import static org.junit.Assert.*;
/**
* CSVParserTest
*
* The test are organized in three different sections:
* The 'setter/getter' section, the lexer section and finally the parser
* section. In case a test fails, you should follow a top-down approach for
* fixing a potential bug (its likely that the parser itself fails if the lexer
* has problems...).
*/
public class CSVParserTest {
String code = "a,b,c,d\n"
+ " a , b , 1 2 \n"
+ "\"foo baar\", b,\n"
// + " \"foo\n,,\n\"\",,\n\\\"\",d,e\n";
+ " \"foo\n,,\n\"\",,\n\"\"\",d,e\n"; // changed to use standard CSV escaping
String[][] res = {
{"a", "b", "c", "d"},
{"a", "b", "1 2"},
{"foo baar", "b", ""},
{"foo\n,,\n\",,\n\"", "d", "e"}
};
@Test
public void testGetLine() throws IOException {
CSVParser parser = new CSVParser(new StringReader(code), CSVFormat.DEFAULT.withSurroundingSpacesIgnored(true));
for (String[] re : res) {
assertArrayEquals(re, parser.getRecord().values());
}
assertNull(parser.getRecord());
}
@Test
public void testGetRecords() throws IOException {
CSVParser parser = new CSVParser(new StringReader(code), CSVFormat.DEFAULT.withSurroundingSpacesIgnored(true));
List<CSVRecord> records = parser.getRecords();
assertEquals(res.length, records.size());
assertTrue(records.size() > 0);
for (int i = 0; i < res.length; i++) {
assertArrayEquals(res[i], records.get(i).values());
}
}
@Test
public void testExcelFormat1() throws IOException {
String code =
"value1,value2,value3,value4\r\na,b,c,d\r\n x,,,"
+ "\r\n\r\n\"\"\"hello\"\"\",\" \"\"world\"\"\",\"abc\ndef\",\r\n";
String[][] res = {
{"value1", "value2", "value3", "value4"},
{"a", "b", "c", "d"},
{" x", "", "", ""},
{""},
{"\"hello\"", " \"world\"", "abc\ndef", ""}
};
CSVParser parser = new CSVParser(code, CSVFormat.EXCEL);
List<CSVRecord> records = parser.getRecords();
assertEquals(res.length, records.size());
assertTrue(records.size() > 0);
for (int i = 0; i < res.length; i++) {
assertArrayEquals(res[i], records.get(i).values());
}
}
@Test
public void testExcelFormat2() throws Exception {
String code = "foo,baar\r\n\r\nhello,\r\n\r\nworld,\r\n";
String[][] res = {
{"foo", "baar"},
{""},
{"hello", ""},
{""},
{"world", ""}
};
CSVParser parser = new CSVParser(code, CSVFormat.EXCEL);
List<CSVRecord> records = parser.getRecords();
assertEquals(res.length, records.size());
assertTrue(records.size() > 0);
for (int i = 0; i < res.length; i++) {
assertArrayEquals(res[i], records.get(i).values());
}
}
@Test
public void testEndOfFileBehaviourExcel() throws Exception {
String[] codes = {
"hello,\r\n\r\nworld,\r\n",
"hello,\r\n\r\nworld,",
"hello,\r\n\r\nworld,\"\"\r\n",
"hello,\r\n\r\nworld,\"\"",
"hello,\r\n\r\nworld,\n",
"hello,\r\n\r\nworld,",
"hello,\r\n\r\nworld,\"\"\n",
"hello,\r\n\r\nworld,\"\""
};
String[][] res = {
{"hello", ""},
{""}, // Excel format does not ignore empty lines
{"world", ""}
};
for (String code : codes) {
CSVParser parser = new CSVParser(code, CSVFormat.EXCEL);
List<CSVRecord> records = parser.getRecords();
assertEquals(res.length, records.size());
assertTrue(records.size() > 0);
for (int i = 0; i < res.length; i++) {
assertArrayEquals(res[i], records.get(i).values());
}
}
}
@Test
public void testEndOfFileBehaviorCSV() throws Exception {
String[] codes = {
"hello,\r\n\r\nworld,\r\n",
"hello,\r\n\r\nworld,",
"hello,\r\n\r\nworld,\"\"\r\n",
"hello,\r\n\r\nworld,\"\"",
"hello,\r\n\r\nworld,\n",
"hello,\r\n\r\nworld,",
"hello,\r\n\r\nworld,\"\"\n",
"hello,\r\n\r\nworld,\"\""
};
String[][] res = {
{"hello", ""}, // CSV format ignores empty lines
{"world", ""}
};
for (String code : codes) {
CSVParser parser = new CSVParser(new StringReader(code));
List<CSVRecord> records = parser.getRecords();
assertEquals(res.length, records.size());
assertTrue(records.size() > 0);
for (int i = 0; i < res.length; i++) {
assertArrayEquals(res[i], records.get(i).values());
}
}
}
@Test
public void testEmptyLineBehaviourExcel() throws Exception {
String[] codes = {
"hello,\r\n\r\n\r\n",
"hello,\n\n\n",
"hello,\"\"\r\n\r\n\r\n",
"hello,\"\"\n\n\n"
};
String[][] res = {
{"hello", ""},
{""}, // Excel format does not ignore empty lines
{""}
};
for (String code : codes) {
CSVParser parser = new CSVParser(code, CSVFormat.EXCEL);
List<CSVRecord> records = parser.getRecords();
assertEquals(res.length, records.size());
assertTrue(records.size() > 0);
for (int i = 0; i < res.length; i++) {
assertArrayEquals(res[i], records.get(i).values());
}
}
}
@Test
public void testEmptyLineBehaviourCSV() throws Exception {
String[] codes = {
"hello,\r\n\r\n\r\n",
"hello,\n\n\n",
"hello,\"\"\r\n\r\n\r\n",
"hello,\"\"\n\n\n"
};
String[][] res = {
{"hello", ""} // CSV format ignores empty lines
};
for (String code : codes) {
CSVParser parser = new CSVParser(new StringReader(code));
List<CSVRecord> records = parser.getRecords();
assertEquals(res.length, records.size());
assertTrue(records.size() > 0);
for (int i = 0; i < res.length; i++) {
assertArrayEquals(res[i], records.get(i).values());
}
}
}
@Test
public void testEmptyFile() throws Exception {
CSVParser parser = new CSVParser("", CSVFormat.DEFAULT);
assertNull(parser.getRecord());
}
@Test
@Ignore
public void testBackslashEscapingOld() throws IOException {
String code =
"one,two,three\n"
+ "on\\\"e,two\n"
+ "on\"e,two\n"
+ "one,\"tw\\\"o\"\n"
+ "one,\"t\\,wo\"\n"
+ "one,two,\"th,ree\"\n"
+ "\"a\\\\\"\n"
+ "a\\,b\n"
+ "\"a\\\\,b\"";
String[][] res = {
{"one", "two", "three"},
{"on\\\"e", "two"},
{"on\"e", "two"},
{"one", "tw\"o"},
{"one", "t\\,wo"}, // backslash in quotes only escapes a delimiter (",")
{"one", "two", "th,ree"},
{"a\\\\"}, // backslash in quotes only escapes a delimiter (",")
{"a\\", "b"}, // a backslash must be returnd
{"a\\\\,b"} // backslash in quotes only escapes a delimiter (",")
};
CSVParser parser = new CSVParser(new StringReader(code));
List<CSVRecord> records = parser.getRecords();
assertEquals(res.length, records.size());
assertTrue(records.size() > 0);
for (int i = 0; i < res.length; i++) {
assertArrayEquals(res[i], records.get(i).values());
}
}
@Test
public void testBackslashEscaping() throws IOException {
// To avoid confusion over the need for escaping chars in java code,
// We will test with a forward slash as the escape char, and a single
// quote as the encapsulator.
String code =
"one,two,three\n" // 0
+ "'',''\n" // 1) empty encapsulators
+ "/',/'\n" // 2) single encapsulators
+ "'/'','/''\n" // 3) single encapsulators encapsulated via escape
+ "'''',''''\n" // 4) single encapsulators encapsulated via doubling
+ "/,,/,\n" // 5) separator escaped
+ "//,//\n" // 6) escape escaped
+ "'//','//'\n" // 7) escape escaped in encapsulation
+ " 8 , \"quoted \"\" /\" // string\" \n" // don't eat spaces
+ "9, /\n \n" // escaped newline
+ "";
String[][] res = {
{"one", "two", "three"}, // 0
{"", ""}, // 1
{"'", "'"}, // 2
{"'", "'"}, // 3
{"'", "'"}, // 4
{",", ","}, // 5
{"/", "/"}, // 6
{"/", "/"}, // 7
{" 8 ", " \"quoted \"\" \" / string\" "},
{"9", " \n "},
};
CSVFormat format = new CSVFormat(',', '\'', CSVFormat.DISABLED, '/', false, true, "\r\n", null);
CSVParser parser = new CSVParser(code, format);
List<CSVRecord> records = parser.getRecords();
assertTrue(records.size() > 0);
for (int i = 0; i < res.length; i++) {
assertArrayEquals(res[i], records.get(i).values());
}
}
@Test
public void testBackslashEscaping2() throws IOException {
// To avoid confusion over the need for escaping chars in java code,
// We will test with a forward slash as the escape char, and a single
// quote as the encapsulator.
String code = ""
+ " , , \n" // 1)
+ " \t , , \n" // 2)
+ " // , /, , /,\n" // 3)
+ "";
String[][] res = {
{" ", " ", " "}, // 1
{" \t ", " ", " "}, // 2
{" / ", " , ", " ,"}, // 3
};
CSVFormat format = new CSVFormat(',', CSVFormat.DISABLED, CSVFormat.DISABLED, '/', false, true, "\r\n", null);
CSVParser parser = new CSVParser(code, format);
List<CSVRecord> records = parser.getRecords();
assertTrue(records.size() > 0);
assertTrue(CSVPrinterTest.equals(res, records));
}
@Test
public void testDefaultFormat() throws IOException {
String code = ""
+ "a,b\n" // 1)
+ "\"\n\",\" \"\n" // 2)
+ "\"\",#\n" // 2)
;
String[][] res = {
{"a", "b"},
{"\n", " "},
{"", "#"},
};
CSVFormat format = CSVFormat.DEFAULT;
assertEquals(CSVFormat.DISABLED, format.getCommentStart());
CSVParser parser = new CSVParser(code, format);
List<CSVRecord> records = parser.getRecords();
assertTrue(records.size() > 0);
assertTrue(CSVPrinterTest.equals(res, records));
String[][] res_comments = {
{"a", "b"},
{"\n", " "},
{""},
};
format = CSVFormat.DEFAULT.withCommentStart('#');
parser = new CSVParser(code, format);
records = parser.getRecords();
assertTrue(CSVPrinterTest.equals(res_comments, records));
}
@Test
public void testCarriageReturnLineFeedEndings() throws IOException {
String code = "foo\r\nbaar,\r\nhello,world\r\n,kanu";
CSVParser parser = new CSVParser(new StringReader(code));
List<CSVRecord> records = parser.getRecords();
assertEquals(4, records.size());
}
@Test
public void testCarriageReturnEndings() throws IOException {
String code = "foo\rbaar,\rhello,world\r,kanu";
CSVParser parser = new CSVParser(new StringReader(code));
List<CSVRecord> records = parser.getRecords();
assertEquals(4, records.size());
}
@Test
public void testLineFeedEndings() throws IOException {
String code = "foo\nbaar,\nhello,world\n,kanu";
CSVParser parser = new CSVParser(new StringReader(code));
List<CSVRecord> records = parser.getRecords();
assertEquals(4, records.size());
}
@Test
public void testIgnoreEmptyLines() throws IOException {
String code = "\nfoo,baar\n\r\n,\n\n,world\r\n\n";
//String code = "world\r\n\n";
//String code = "foo;baar\r\n\r\nhello;\r\n\r\nworld;\r\n";
CSVParser parser = new CSVParser(new StringReader(code));
List<CSVRecord> records = parser.getRecords();
assertEquals(3, records.size());
}
@Test
public void testForEach() throws Exception {
List<CSVRecord> records = new ArrayList<CSVRecord>();
Reader in = new StringReader("a,b,c\n1,2,3\nx,y,z");
for (CSVRecord record : CSVFormat.DEFAULT.parse(in)) {
records.add(record);
}
assertEquals(3, records.size());
assertArrayEquals(new String[]{"a", "b", "c"}, records.get(0).values());
assertArrayEquals(new String[]{"1", "2", "3"}, records.get(1).values());
assertArrayEquals(new String[]{"x", "y", "z"}, records.get(2).values());
}
@Test
public void testIterator() throws Exception {
Reader in = new StringReader("a,b,c\n1,2,3\nx,y,z");
Iterator<CSVRecord> iterator = CSVFormat.DEFAULT.parse(in).iterator();
assertTrue(iterator.hasNext());
try {
iterator.remove();
fail("expected UnsupportedOperationException");
} catch (UnsupportedOperationException expected) {
}
assertArrayEquals(new String[]{"a", "b", "c"}, iterator.next().values());
assertArrayEquals(new String[]{"1", "2", "3"}, iterator.next().values());
assertTrue(iterator.hasNext());
assertTrue(iterator.hasNext());
assertTrue(iterator.hasNext());
assertArrayEquals(new String[]{"x", "y", "z"}, iterator.next().values());
assertFalse(iterator.hasNext());
try {
iterator.next();
fail("NoSuchElementException expected");
} catch (NoSuchElementException e) {
// expected
}
}
@Test
public void testHeader() throws Exception {
Reader in = new StringReader("a,b,c\n1,2,3\nx,y,z");
Iterator<CSVRecord> records = CSVFormat.DEFAULT.withHeader().parse(in).iterator();
for (int i = 0; i < 2; i++) {
assertTrue(records.hasNext());
CSVRecord record = records.next();
assertEquals(record.get(0), record.get("a"));
assertEquals(record.get(1), record.get("b"));
assertEquals(record.get(2), record.get("c"));
}
assertFalse(records.hasNext());
}
@Test
public void testProvidedHeader() throws Exception {
Reader in = new StringReader("a,b,c\n1,2,3\nx,y,z");
Iterator<CSVRecord> records = CSVFormat.DEFAULT.withHeader("A", "B", "C").parse(in).iterator();
for (int i = 0; i < 3; i++) {
assertTrue(records.hasNext());
CSVRecord record = records.next();
assertEquals(record.get(0), record.get("A"));
assertEquals(record.get(1), record.get("B"));
assertEquals(record.get(2), record.get("C"));
}
assertFalse(records.hasNext());
}
@Test
public void testGetLineNumberWithLF() throws Exception {
CSVParser parser = new CSVParser("a\nb\nc", CSVFormat.DEFAULT.withLineSeparator("\n"));
assertEquals(0, parser.getLineNumber());
assertNotNull(parser.getRecord());
assertEquals(1, parser.getLineNumber());
assertNotNull(parser.getRecord());
assertEquals(2, parser.getLineNumber());
assertNotNull(parser.getRecord());
assertEquals(2, parser.getLineNumber());
assertNull(parser.getRecord());
}
@Test
public void testGetLineNumberWithCRLF() throws Exception {
CSVParser parser = new CSVParser("a\r\nb\r\nc", CSVFormat.DEFAULT.withLineSeparator("\r\n"));
assertEquals(0, parser.getLineNumber());
assertNotNull(parser.getRecord());
assertEquals(1, parser.getLineNumber());
assertNotNull(parser.getRecord());
assertEquals(2, parser.getLineNumber());
assertNotNull(parser.getRecord());
assertEquals(2, parser.getLineNumber());
assertNull(parser.getRecord());
}
@Test
public void testGetLineNumberWithCR() throws Exception {
CSVParser parser = new CSVParser("a\rb\rc", CSVFormat.DEFAULT.withLineSeparator("\r"));
assertEquals(0, parser.getLineNumber());
assertNotNull(parser.getRecord());
assertEquals(1, parser.getLineNumber());
assertNotNull(parser.getRecord());
assertEquals(2, parser.getLineNumber());
assertNotNull(parser.getRecord());
assertEquals(2, parser.getLineNumber());
assertNull(parser.getRecord());
}
} |
||
public void testLocationOffsets() throws Exception
{
JsonParser parser = DEFAULT_F.createNonBlockingByteArrayParser();
ByteArrayFeeder feeder = (ByteArrayFeeder) parser.getNonBlockingInputFeeder();
byte[] input = utf8Bytes("[[[");
feeder.feedInput(input, 2, 3);
assertEquals(JsonToken.START_ARRAY, parser.nextToken());
assertEquals(1, parser.getCurrentLocation().getByteOffset());
assertEquals(1, parser.getTokenLocation().getByteOffset());
assertEquals(1, parser.getCurrentLocation().getLineNr());
assertEquals(1, parser.getTokenLocation().getLineNr());
assertEquals(2, parser.getCurrentLocation().getColumnNr());
assertEquals(1, parser.getTokenLocation().getColumnNr());
feeder.feedInput(input, 0, 1);
assertEquals(JsonToken.START_ARRAY, parser.nextToken());
assertEquals(2, parser.getCurrentLocation().getByteOffset());
assertEquals(2, parser.getTokenLocation().getByteOffset());
assertEquals(1, parser.getCurrentLocation().getLineNr());
assertEquals(1, parser.getTokenLocation().getLineNr());
assertEquals(3, parser.getCurrentLocation().getColumnNr());
assertEquals(2, parser.getTokenLocation().getColumnNr());
parser.close();
} | com.fasterxml.jackson.core.json.async.AsyncLocationTest::testLocationOffsets | src/test/java/com/fasterxml/jackson/core/json/async/AsyncLocationTest.java | 36 | src/test/java/com/fasterxml/jackson/core/json/async/AsyncLocationTest.java | testLocationOffsets | package com.fasterxml.jackson.core.json.async;
import com.fasterxml.jackson.core.*;
import com.fasterxml.jackson.core.async.AsyncTestBase;
import com.fasterxml.jackson.core.async.ByteArrayFeeder;
public class AsyncLocationTest extends AsyncTestBase
{
private final JsonFactory DEFAULT_F = new JsonFactory();
// for [core#531]
public void testLocationOffsets() throws Exception
{
JsonParser parser = DEFAULT_F.createNonBlockingByteArrayParser();
ByteArrayFeeder feeder = (ByteArrayFeeder) parser.getNonBlockingInputFeeder();
byte[] input = utf8Bytes("[[[");
feeder.feedInput(input, 2, 3);
assertEquals(JsonToken.START_ARRAY, parser.nextToken());
assertEquals(1, parser.getCurrentLocation().getByteOffset());
assertEquals(1, parser.getTokenLocation().getByteOffset());
assertEquals(1, parser.getCurrentLocation().getLineNr());
assertEquals(1, parser.getTokenLocation().getLineNr());
assertEquals(2, parser.getCurrentLocation().getColumnNr());
assertEquals(1, parser.getTokenLocation().getColumnNr());
feeder.feedInput(input, 0, 1);
assertEquals(JsonToken.START_ARRAY, parser.nextToken());
assertEquals(2, parser.getCurrentLocation().getByteOffset());
assertEquals(2, parser.getTokenLocation().getByteOffset());
assertEquals(1, parser.getCurrentLocation().getLineNr());
assertEquals(1, parser.getTokenLocation().getLineNr());
assertEquals(3, parser.getCurrentLocation().getColumnNr());
assertEquals(2, parser.getTokenLocation().getColumnNr());
parser.close();
}
} | // You are a professional Java test case writer, please create a test case named `testLocationOffsets` for the issue `JacksonCore-531`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonCore-531
//
// ## Issue-Title:
// Non-blocking parser reports incorrect locations when fed with non-zero offset
//
// ## Issue-Description:
// When feeding a non-blocking parser, the input array offset leaks into the offsets reported by `getCurrentLocation()` and `getTokenLocation()`.
//
//
// For example, feeding with an offset of 7 yields tokens whose reported locations are 7 greater than they should be. Likewise the current location reported by the parser is 7 greater than the correct location.
//
//
// It's not possible for a user to work around this issue by subtracting 7 from the reported locations, because the token location may have been established by an earlier feeding with a different offset.
//
//
// Jackson version: 2.9.8
//
//
// Unit test:
//
//
//
// ```
// import com.fasterxml.jackson.core.JsonFactory;
// import com.fasterxml.jackson.core.JsonParser;
// import com.fasterxml.jackson.core.JsonToken;
// import com.fasterxml.jackson.core.async.ByteArrayFeeder;
// import org.junit.Test;
//
// import static java.nio.charset.StandardCharsets.UTF\_8;
// import static org.junit.Assert.assertEquals;
//
// public class FeedingOffsetTest {
//
// @Test
// public void inputOffsetShouldNotAffectLocations() throws Exception {
// JsonFactory jsonFactory = new JsonFactory();
// JsonParser parser = jsonFactory.createNonBlockingByteArrayParser();
// ByteArrayFeeder feeder = (ByteArrayFeeder) parser.getNonBlockingInputFeeder();
//
// byte[] input = "[[[".getBytes(UTF\_8);
//
// feeder.feedInput(input, 2, 3);
// assertEquals(JsonToken.START\_ARRAY, parser.nextToken());
// assertEquals(1, parser.getCurrentLocation().getByteOffset()); // ACTUAL = 3
// assertEquals(1, parser.getTokenLocation().getByteOffset()); // ACTUAL = 3
//
// feeder.feedInput(input, 0, 1);
// assertEquals(JsonToken.START\_ARRAY, parser.nextToken());
// assertEquals(2, parser.getCurrentLocation().getByteOffset());
// assertEquals(2, parser.getTokenLocation().getByteOffset());
// }
// }
// ```
//
//
//
public void testLocationOffsets() throws Exception {
| 36 | // for [core#531] | 26 | 11 | src/test/java/com/fasterxml/jackson/core/json/async/AsyncLocationTest.java | src/test/java | ```markdown
## Issue-ID: JacksonCore-531
## Issue-Title:
Non-blocking parser reports incorrect locations when fed with non-zero offset
## Issue-Description:
When feeding a non-blocking parser, the input array offset leaks into the offsets reported by `getCurrentLocation()` and `getTokenLocation()`.
For example, feeding with an offset of 7 yields tokens whose reported locations are 7 greater than they should be. Likewise the current location reported by the parser is 7 greater than the correct location.
It's not possible for a user to work around this issue by subtracting 7 from the reported locations, because the token location may have been established by an earlier feeding with a different offset.
Jackson version: 2.9.8
Unit test:
```
import com.fasterxml.jackson.core.JsonFactory;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.core.JsonToken;
import com.fasterxml.jackson.core.async.ByteArrayFeeder;
import org.junit.Test;
import static java.nio.charset.StandardCharsets.UTF\_8;
import static org.junit.Assert.assertEquals;
public class FeedingOffsetTest {
@Test
public void inputOffsetShouldNotAffectLocations() throws Exception {
JsonFactory jsonFactory = new JsonFactory();
JsonParser parser = jsonFactory.createNonBlockingByteArrayParser();
ByteArrayFeeder feeder = (ByteArrayFeeder) parser.getNonBlockingInputFeeder();
byte[] input = "[[[".getBytes(UTF\_8);
feeder.feedInput(input, 2, 3);
assertEquals(JsonToken.START\_ARRAY, parser.nextToken());
assertEquals(1, parser.getCurrentLocation().getByteOffset()); // ACTUAL = 3
assertEquals(1, parser.getTokenLocation().getByteOffset()); // ACTUAL = 3
feeder.feedInput(input, 0, 1);
assertEquals(JsonToken.START\_ARRAY, parser.nextToken());
assertEquals(2, parser.getCurrentLocation().getByteOffset());
assertEquals(2, parser.getTokenLocation().getByteOffset());
}
}
```
```
You are a professional Java test case writer, please create a test case named `testLocationOffsets` for the issue `JacksonCore-531`, utilizing the provided issue report information and the following function signature.
```java
public void testLocationOffsets() throws Exception {
```
| 11 | [
"com.fasterxml.jackson.core.json.async.NonBlockingJsonParser"
] | 74cf61b24d9566ce3276db992a55297d5b3bbbaf156801bea4904f69aa3bf8dc | public void testLocationOffsets() throws Exception
| // You are a professional Java test case writer, please create a test case named `testLocationOffsets` for the issue `JacksonCore-531`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonCore-531
//
// ## Issue-Title:
// Non-blocking parser reports incorrect locations when fed with non-zero offset
//
// ## Issue-Description:
// When feeding a non-blocking parser, the input array offset leaks into the offsets reported by `getCurrentLocation()` and `getTokenLocation()`.
//
//
// For example, feeding with an offset of 7 yields tokens whose reported locations are 7 greater than they should be. Likewise the current location reported by the parser is 7 greater than the correct location.
//
//
// It's not possible for a user to work around this issue by subtracting 7 from the reported locations, because the token location may have been established by an earlier feeding with a different offset.
//
//
// Jackson version: 2.9.8
//
//
// Unit test:
//
//
//
// ```
// import com.fasterxml.jackson.core.JsonFactory;
// import com.fasterxml.jackson.core.JsonParser;
// import com.fasterxml.jackson.core.JsonToken;
// import com.fasterxml.jackson.core.async.ByteArrayFeeder;
// import org.junit.Test;
//
// import static java.nio.charset.StandardCharsets.UTF\_8;
// import static org.junit.Assert.assertEquals;
//
// public class FeedingOffsetTest {
//
// @Test
// public void inputOffsetShouldNotAffectLocations() throws Exception {
// JsonFactory jsonFactory = new JsonFactory();
// JsonParser parser = jsonFactory.createNonBlockingByteArrayParser();
// ByteArrayFeeder feeder = (ByteArrayFeeder) parser.getNonBlockingInputFeeder();
//
// byte[] input = "[[[".getBytes(UTF\_8);
//
// feeder.feedInput(input, 2, 3);
// assertEquals(JsonToken.START\_ARRAY, parser.nextToken());
// assertEquals(1, parser.getCurrentLocation().getByteOffset()); // ACTUAL = 3
// assertEquals(1, parser.getTokenLocation().getByteOffset()); // ACTUAL = 3
//
// feeder.feedInput(input, 0, 1);
// assertEquals(JsonToken.START\_ARRAY, parser.nextToken());
// assertEquals(2, parser.getCurrentLocation().getByteOffset());
// assertEquals(2, parser.getTokenLocation().getByteOffset());
// }
// }
// ```
//
//
//
| JacksonCore | package com.fasterxml.jackson.core.json.async;
import com.fasterxml.jackson.core.*;
import com.fasterxml.jackson.core.async.AsyncTestBase;
import com.fasterxml.jackson.core.async.ByteArrayFeeder;
public class AsyncLocationTest extends AsyncTestBase
{
private final JsonFactory DEFAULT_F = new JsonFactory();
// for [core#531]
public void testLocationOffsets() throws Exception
{
JsonParser parser = DEFAULT_F.createNonBlockingByteArrayParser();
ByteArrayFeeder feeder = (ByteArrayFeeder) parser.getNonBlockingInputFeeder();
byte[] input = utf8Bytes("[[[");
feeder.feedInput(input, 2, 3);
assertEquals(JsonToken.START_ARRAY, parser.nextToken());
assertEquals(1, parser.getCurrentLocation().getByteOffset());
assertEquals(1, parser.getTokenLocation().getByteOffset());
assertEquals(1, parser.getCurrentLocation().getLineNr());
assertEquals(1, parser.getTokenLocation().getLineNr());
assertEquals(2, parser.getCurrentLocation().getColumnNr());
assertEquals(1, parser.getTokenLocation().getColumnNr());
feeder.feedInput(input, 0, 1);
assertEquals(JsonToken.START_ARRAY, parser.nextToken());
assertEquals(2, parser.getCurrentLocation().getByteOffset());
assertEquals(2, parser.getTokenLocation().getByteOffset());
assertEquals(1, parser.getCurrentLocation().getLineNr());
assertEquals(1, parser.getTokenLocation().getLineNr());
assertEquals(3, parser.getCurrentLocation().getColumnNr());
assertEquals(2, parser.getTokenLocation().getColumnNr());
parser.close();
}
} |
|
public void testWonkyNumber173() throws Exception
{
JsonPointer ptr = JsonPointer.compile("/1e0");
assertFalse(ptr.matches());
} | com.fasterxml.jackson.core.TestJsonPointer::testWonkyNumber173 | src/test/java/com/fasterxml/jackson/core/TestJsonPointer.java | 42 | src/test/java/com/fasterxml/jackson/core/TestJsonPointer.java | testWonkyNumber173 | package com.fasterxml.jackson.core;
import com.fasterxml.jackson.test.BaseTest;
public class TestJsonPointer extends BaseTest
{
public void testSimplePath() throws Exception
{
final String INPUT = "/Image/15/name";
JsonPointer ptr = JsonPointer.compile(INPUT);
assertFalse(ptr.matches());
assertEquals(-1, ptr.getMatchingIndex());
assertEquals("Image", ptr.getMatchingProperty());
assertEquals(INPUT, ptr.toString());
ptr = ptr.tail();
assertNotNull(ptr);
assertFalse(ptr.matches());
assertEquals(15, ptr.getMatchingIndex());
assertEquals("15", ptr.getMatchingProperty());
assertEquals("/15/name", ptr.toString());
ptr = ptr.tail();
assertNotNull(ptr);
assertFalse(ptr.matches());
assertEquals(-1, ptr.getMatchingIndex());
assertEquals("name", ptr.getMatchingProperty());
assertEquals("/name", ptr.toString());
// done!
ptr = ptr.tail();
assertTrue(ptr.matches());
assertNull(ptr.tail());
assertEquals("", ptr.getMatchingProperty());
assertEquals(-1, ptr.getMatchingIndex());
}
public void testWonkyNumber173() throws Exception
{
JsonPointer ptr = JsonPointer.compile("/1e0");
assertFalse(ptr.matches());
}
public void testQuotedPath() throws Exception
{
final String INPUT = "/w~1out/til~0de/a~1b";
JsonPointer ptr = JsonPointer.compile(INPUT);
assertFalse(ptr.matches());
assertEquals(-1, ptr.getMatchingIndex());
assertEquals("w/out", ptr.getMatchingProperty());
assertEquals(INPUT, ptr.toString());
ptr = ptr.tail();
assertNotNull(ptr);
assertFalse(ptr.matches());
assertEquals(-1, ptr.getMatchingIndex());
assertEquals("til~de", ptr.getMatchingProperty());
assertEquals("/til~0de/a~1b", ptr.toString());
ptr = ptr.tail();
assertNotNull(ptr);
assertFalse(ptr.matches());
assertEquals(-1, ptr.getMatchingIndex());
assertEquals("a/b", ptr.getMatchingProperty());
assertEquals("/a~1b", ptr.toString());
// done!
ptr = ptr.tail();
assertTrue(ptr.matches());
assertNull(ptr.tail());
}
// [Issue#133]
public void testLongNumbers() throws Exception
{
final long LONG_ID = ((long) Integer.MAX_VALUE) + 1L;
final String INPUT = "/User/"+LONG_ID;
JsonPointer ptr = JsonPointer.compile(INPUT);
assertEquals("User", ptr.getMatchingProperty());
assertEquals(INPUT, ptr.toString());
ptr = ptr.tail();
assertNotNull(ptr);
assertFalse(ptr.matches());
/* 14-Mar-2014, tatu: We do not support array indexes beyond 32-bit
* range; can still match textually of course.
*/
assertEquals(-1, ptr.getMatchingIndex());
assertEquals(String.valueOf(LONG_ID), ptr.getMatchingProperty());
// done!
ptr = ptr.tail();
assertTrue(ptr.matches());
assertNull(ptr.tail());
}
} | // You are a professional Java test case writer, please create a test case named `testWonkyNumber173` for the issue `JacksonCore-173`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonCore-173
//
// ## Issue-Title:
// An exception is thrown for a valid JsonPointer expression
//
// ## Issue-Description:
// Json-Patch project leader has noted me that there is a bug on JsonPointer implementation and I have decided to investigate.
//
//
// Basically if you do something like `JsonPointer.compile("/1e0");` it throws a NumberFormatExpcetion which is not true. This is because this piece of code:
//
//
//
// ```
// private final static int \_parseInt(String str)
// {
// final int len = str.length();
// if (len == 0) {
// return -1;
// }
// for (int i = 0; i < len; ++i) {
// char c = str.charAt(i++);
// if (c > '9' || c < '0') {
// return -1;
// }
// }
// // for now, we'll assume 32-bit indexes are fine
// return NumberInput.parseInt(str);
// }
// ```
//
// When they found a number it interprets the segment as integer but in reality it should be the whole expression. For this reason I think that the condition should be changed to the inverse condition (if it doesn't found any char then it is a number.
//
//
// If you want I can send you a PR as well.
//
//
// Alex.
//
//
//
//
public void testWonkyNumber173() throws Exception {
| 42 | 5 | 38 | src/test/java/com/fasterxml/jackson/core/TestJsonPointer.java | src/test/java | ```markdown
## Issue-ID: JacksonCore-173
## Issue-Title:
An exception is thrown for a valid JsonPointer expression
## Issue-Description:
Json-Patch project leader has noted me that there is a bug on JsonPointer implementation and I have decided to investigate.
Basically if you do something like `JsonPointer.compile("/1e0");` it throws a NumberFormatExpcetion which is not true. This is because this piece of code:
```
private final static int \_parseInt(String str)
{
final int len = str.length();
if (len == 0) {
return -1;
}
for (int i = 0; i < len; ++i) {
char c = str.charAt(i++);
if (c > '9' || c < '0') {
return -1;
}
}
// for now, we'll assume 32-bit indexes are fine
return NumberInput.parseInt(str);
}
```
When they found a number it interprets the segment as integer but in reality it should be the whole expression. For this reason I think that the condition should be changed to the inverse condition (if it doesn't found any char then it is a number.
If you want I can send you a PR as well.
Alex.
```
You are a professional Java test case writer, please create a test case named `testWonkyNumber173` for the issue `JacksonCore-173`, utilizing the provided issue report information and the following function signature.
```java
public void testWonkyNumber173() throws Exception {
```
| 38 | [
"com.fasterxml.jackson.core.JsonPointer"
] | 754589f118a4d5c9560555ac6418741d914788c077610a8d03bdb59994623ee3 | public void testWonkyNumber173() throws Exception
| // You are a professional Java test case writer, please create a test case named `testWonkyNumber173` for the issue `JacksonCore-173`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonCore-173
//
// ## Issue-Title:
// An exception is thrown for a valid JsonPointer expression
//
// ## Issue-Description:
// Json-Patch project leader has noted me that there is a bug on JsonPointer implementation and I have decided to investigate.
//
//
// Basically if you do something like `JsonPointer.compile("/1e0");` it throws a NumberFormatExpcetion which is not true. This is because this piece of code:
//
//
//
// ```
// private final static int \_parseInt(String str)
// {
// final int len = str.length();
// if (len == 0) {
// return -1;
// }
// for (int i = 0; i < len; ++i) {
// char c = str.charAt(i++);
// if (c > '9' || c < '0') {
// return -1;
// }
// }
// // for now, we'll assume 32-bit indexes are fine
// return NumberInput.parseInt(str);
// }
// ```
//
// When they found a number it interprets the segment as integer but in reality it should be the whole expression. For this reason I think that the condition should be changed to the inverse condition (if it doesn't found any char then it is a number.
//
//
// If you want I can send you a PR as well.
//
//
// Alex.
//
//
//
//
| JacksonCore | package com.fasterxml.jackson.core;
import com.fasterxml.jackson.test.BaseTest;
public class TestJsonPointer extends BaseTest
{
public void testSimplePath() throws Exception
{
final String INPUT = "/Image/15/name";
JsonPointer ptr = JsonPointer.compile(INPUT);
assertFalse(ptr.matches());
assertEquals(-1, ptr.getMatchingIndex());
assertEquals("Image", ptr.getMatchingProperty());
assertEquals(INPUT, ptr.toString());
ptr = ptr.tail();
assertNotNull(ptr);
assertFalse(ptr.matches());
assertEquals(15, ptr.getMatchingIndex());
assertEquals("15", ptr.getMatchingProperty());
assertEquals("/15/name", ptr.toString());
ptr = ptr.tail();
assertNotNull(ptr);
assertFalse(ptr.matches());
assertEquals(-1, ptr.getMatchingIndex());
assertEquals("name", ptr.getMatchingProperty());
assertEquals("/name", ptr.toString());
// done!
ptr = ptr.tail();
assertTrue(ptr.matches());
assertNull(ptr.tail());
assertEquals("", ptr.getMatchingProperty());
assertEquals(-1, ptr.getMatchingIndex());
}
public void testWonkyNumber173() throws Exception
{
JsonPointer ptr = JsonPointer.compile("/1e0");
assertFalse(ptr.matches());
}
public void testQuotedPath() throws Exception
{
final String INPUT = "/w~1out/til~0de/a~1b";
JsonPointer ptr = JsonPointer.compile(INPUT);
assertFalse(ptr.matches());
assertEquals(-1, ptr.getMatchingIndex());
assertEquals("w/out", ptr.getMatchingProperty());
assertEquals(INPUT, ptr.toString());
ptr = ptr.tail();
assertNotNull(ptr);
assertFalse(ptr.matches());
assertEquals(-1, ptr.getMatchingIndex());
assertEquals("til~de", ptr.getMatchingProperty());
assertEquals("/til~0de/a~1b", ptr.toString());
ptr = ptr.tail();
assertNotNull(ptr);
assertFalse(ptr.matches());
assertEquals(-1, ptr.getMatchingIndex());
assertEquals("a/b", ptr.getMatchingProperty());
assertEquals("/a~1b", ptr.toString());
// done!
ptr = ptr.tail();
assertTrue(ptr.matches());
assertNull(ptr.tail());
}
// [Issue#133]
public void testLongNumbers() throws Exception
{
final long LONG_ID = ((long) Integer.MAX_VALUE) + 1L;
final String INPUT = "/User/"+LONG_ID;
JsonPointer ptr = JsonPointer.compile(INPUT);
assertEquals("User", ptr.getMatchingProperty());
assertEquals(INPUT, ptr.toString());
ptr = ptr.tail();
assertNotNull(ptr);
assertFalse(ptr.matches());
/* 14-Mar-2014, tatu: We do not support array indexes beyond 32-bit
* range; can still match textually of course.
*/
assertEquals(-1, ptr.getMatchingIndex());
assertEquals(String.valueOf(LONG_ID), ptr.getMatchingProperty());
// done!
ptr = ptr.tail();
assertTrue(ptr.matches());
assertNull(ptr.tail());
}
} |
||
public void testLang328() {
assertValidToLocale("fr__POSIX", "fr", "", "POSIX");
} | org.apache.commons.lang.LocaleUtilsTest::testLang328 | src/test/org/apache/commons/lang/LocaleUtilsTest.java | 505 | src/test/org/apache/commons/lang/LocaleUtilsTest.java | testLang328 | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.lang;
import java.lang.reflect.Constructor;
import java.lang.reflect.Modifier;
import java.util.Arrays;
import java.util.Collection;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Locale;
import java.util.Set;
import junit.framework.Test;
import junit.framework.TestCase;
import junit.framework.TestSuite;
import junit.textui.TestRunner;
/**
* Unit tests for {@link LocaleUtils}.
*
* @author Chris Hyzer
* @author Stephen Colebourne
* @version $Id$
*/
public class LocaleUtilsTest extends TestCase {
private static final Locale LOCALE_EN = new Locale("en", "");
private static final Locale LOCALE_EN_US = new Locale("en", "US");
private static final Locale LOCALE_EN_US_ZZZZ = new Locale("en", "US", "ZZZZ");
private static final Locale LOCALE_FR = new Locale("fr", "");
private static final Locale LOCALE_FR_CA = new Locale("fr", "CA");
private static final Locale LOCALE_QQ = new Locale("qq", "");
private static final Locale LOCALE_QQ_ZZ = new Locale("qq", "ZZ");
/**
* Constructor.
*
* @param name
*/
public LocaleUtilsTest(String name) {
super(name);
}
/**
* Main.
* @param args
*/
public static void main(String[] args) {
TestRunner.run(suite());
}
/**
* Run the test cases as a suite.
* @return the Test
*/
public static Test suite() {
TestSuite suite = new TestSuite(LocaleUtilsTest.class);
suite.setName("LocaleUtilsTest Tests");
return suite;
}
public void setUp() throws Exception {
super.setUp();
// Testing #LANG-304. Must be called before availableLocaleSet is called.
LocaleUtils.isAvailableLocale(Locale.getDefault());
}
//-----------------------------------------------------------------------
/**
* Test that constructors are public, and work, etc.
*/
public void testConstructor() {
assertNotNull(new LocaleUtils());
Constructor[] cons = LocaleUtils.class.getDeclaredConstructors();
assertEquals(1, cons.length);
assertEquals(true, Modifier.isPublic(cons[0].getModifiers()));
assertEquals(true, Modifier.isPublic(LocaleUtils.class.getModifiers()));
assertEquals(false, Modifier.isFinal(LocaleUtils.class.getModifiers()));
}
//-----------------------------------------------------------------------
/**
* Pass in a valid language, test toLocale.
*
* @param language the language string
*/
private void assertValidToLocale(String language) {
Locale locale = LocaleUtils.toLocale(language);
assertNotNull("valid locale", locale);
assertEquals(language, locale.getLanguage());
//country and variant are empty
assertTrue(locale.getCountry() == null || locale.getCountry().length() == 0);
assertTrue(locale.getVariant() == null || locale.getVariant().length() == 0);
}
/**
* Pass in a valid language, test toLocale.
*
* @param localeString to pass to toLocale()
* @param language of the resulting Locale
* @param country of the resulting Locale
*/
private void assertValidToLocale(String localeString, String language, String country) {
Locale locale = LocaleUtils.toLocale(localeString);
assertNotNull("valid locale", locale);
assertEquals(language, locale.getLanguage());
assertEquals(country, locale.getCountry());
//variant is empty
assertTrue(locale.getVariant() == null || locale.getVariant().length() == 0);
}
/**
* Pass in a valid language, test toLocale.
*
* @param localeString to pass to toLocale()
* @param language of the resulting Locale
* @param country of the resulting Locale
* @param variant of the resulting Locale
*/
private void assertValidToLocale(
String localeString, String language,
String country, String variant) {
Locale locale = LocaleUtils.toLocale(localeString);
assertNotNull("valid locale", locale);
assertEquals(language, locale.getLanguage());
assertEquals(country, locale.getCountry());
assertEquals(variant, locale.getVariant());
}
/**
* Test toLocale() method.
*/
public void testToLocale_1Part() {
assertEquals(null, LocaleUtils.toLocale((String) null));
assertValidToLocale("us");
assertValidToLocale("fr");
assertValidToLocale("de");
assertValidToLocale("zh");
// Valid format but lang doesnt exist, should make instance anyway
assertValidToLocale("qq");
try {
LocaleUtils.toLocale("Us");
fail("Should fail if not lowercase");
} catch (IllegalArgumentException iae) {}
try {
LocaleUtils.toLocale("US");
fail("Should fail if not lowercase");
} catch (IllegalArgumentException iae) {}
try {
LocaleUtils.toLocale("uS");
fail("Should fail if not lowercase");
} catch (IllegalArgumentException iae) {}
try {
LocaleUtils.toLocale("u#");
fail("Should fail if not lowercase");
} catch (IllegalArgumentException iae) {}
try {
LocaleUtils.toLocale("u");
fail("Must be 2 chars if less than 5");
} catch (IllegalArgumentException iae) {}
try {
LocaleUtils.toLocale("uuu");
fail("Must be 2 chars if less than 5");
} catch (IllegalArgumentException iae) {}
try {
LocaleUtils.toLocale("uu_U");
fail("Must be 2 chars if less than 5");
} catch (IllegalArgumentException iae) {}
}
/**
* Test toLocale() method.
*/
public void testToLocale_2Part() {
assertValidToLocale("us_EN", "us", "EN");
//valid though doesnt exist
assertValidToLocale("us_ZH", "us", "ZH");
try {
LocaleUtils.toLocale("us-EN");
fail("Should fail as not underscore");
} catch (IllegalArgumentException iae) {}
try {
LocaleUtils.toLocale("us_En");
fail("Should fail second part not uppercase");
} catch (IllegalArgumentException iae) {}
try {
LocaleUtils.toLocale("us_en");
fail("Should fail second part not uppercase");
} catch (IllegalArgumentException iae) {}
try {
LocaleUtils.toLocale("us_eN");
fail("Should fail second part not uppercase");
} catch (IllegalArgumentException iae) {}
try {
LocaleUtils.toLocale("uS_EN");
fail("Should fail first part not lowercase");
} catch (IllegalArgumentException iae) {}
try {
LocaleUtils.toLocale("us_E3");
fail("Should fail second part not uppercase");
} catch (IllegalArgumentException iae) {}
}
/**
* Test toLocale() method.
*/
public void testToLocale_3Part() {
assertValidToLocale("us_EN_A", "us", "EN", "A");
// this isn't pretty, but was caused by a jdk bug it seems
// http://bugs.sun.com/bugdatabase/view_bug.do?bug_id=4210525
if (SystemUtils.isJavaVersionAtLeast(1.4f)) {
assertValidToLocale("us_EN_a", "us", "EN", "a");
assertValidToLocale("us_EN_SFsafdFDsdfF", "us", "EN", "SFsafdFDsdfF");
} else {
assertValidToLocale("us_EN_a", "us", "EN", "A");
assertValidToLocale("us_EN_SFsafdFDsdfF", "us", "EN", "SFSAFDFDSDFF");
}
try {
LocaleUtils.toLocale("us_EN-a");
fail("Should fail as not underscore");
} catch (IllegalArgumentException iae) {}
try {
LocaleUtils.toLocale("uu_UU_");
fail("Must be 3, 5 or 7+ in length");
} catch (IllegalArgumentException iae) {}
}
//-----------------------------------------------------------------------
/**
* Helper method for local lookups.
*
* @param locale the input locale
* @param defaultLocale the input default locale
* @param expected expected results
*/
private void assertLocaleLookupList(Locale locale, Locale defaultLocale, Locale[] expected) {
List localeList = defaultLocale == null ?
LocaleUtils.localeLookupList(locale) :
LocaleUtils.localeLookupList(locale, defaultLocale);
assertEquals(expected.length, localeList.size());
assertEquals(Arrays.asList(expected), localeList);
assertUnmodifiableCollection(localeList);
}
//-----------------------------------------------------------------------
/**
* Test localeLookupList() method.
*/
public void testLocaleLookupList_Locale() {
assertLocaleLookupList(null, null, new Locale[0]);
assertLocaleLookupList(LOCALE_QQ, null, new Locale[]{LOCALE_QQ});
assertLocaleLookupList(LOCALE_EN, null, new Locale[]{LOCALE_EN});
assertLocaleLookupList(LOCALE_EN, null, new Locale[]{LOCALE_EN});
assertLocaleLookupList(LOCALE_EN_US, null,
new Locale[] {
LOCALE_EN_US,
LOCALE_EN});
assertLocaleLookupList(LOCALE_EN_US_ZZZZ, null,
new Locale[] {
LOCALE_EN_US_ZZZZ,
LOCALE_EN_US,
LOCALE_EN});
}
/**
* Test localeLookupList() method.
*/
public void testLocaleLookupList_LocaleLocale() {
assertLocaleLookupList(LOCALE_QQ, LOCALE_QQ,
new Locale[]{LOCALE_QQ});
assertLocaleLookupList(LOCALE_EN, LOCALE_EN,
new Locale[]{LOCALE_EN});
assertLocaleLookupList(LOCALE_EN_US, LOCALE_EN_US,
new Locale[]{
LOCALE_EN_US,
LOCALE_EN});
assertLocaleLookupList(LOCALE_EN_US, LOCALE_QQ,
new Locale[] {
LOCALE_EN_US,
LOCALE_EN,
LOCALE_QQ});
assertLocaleLookupList(LOCALE_EN_US, LOCALE_QQ_ZZ,
new Locale[] {
LOCALE_EN_US,
LOCALE_EN,
LOCALE_QQ_ZZ});
assertLocaleLookupList(LOCALE_EN_US_ZZZZ, null,
new Locale[] {
LOCALE_EN_US_ZZZZ,
LOCALE_EN_US,
LOCALE_EN});
assertLocaleLookupList(LOCALE_EN_US_ZZZZ, LOCALE_EN_US_ZZZZ,
new Locale[] {
LOCALE_EN_US_ZZZZ,
LOCALE_EN_US,
LOCALE_EN});
assertLocaleLookupList(LOCALE_EN_US_ZZZZ, LOCALE_QQ,
new Locale[] {
LOCALE_EN_US_ZZZZ,
LOCALE_EN_US,
LOCALE_EN,
LOCALE_QQ});
assertLocaleLookupList(LOCALE_EN_US_ZZZZ, LOCALE_QQ_ZZ,
new Locale[] {
LOCALE_EN_US_ZZZZ,
LOCALE_EN_US,
LOCALE_EN,
LOCALE_QQ_ZZ});
assertLocaleLookupList(LOCALE_FR_CA, LOCALE_EN,
new Locale[] {
LOCALE_FR_CA,
LOCALE_FR,
LOCALE_EN});
}
//-----------------------------------------------------------------------
/**
* Test availableLocaleList() method.
*/
public void testAvailableLocaleList() {
List list = LocaleUtils.availableLocaleList();
List list2 = LocaleUtils.availableLocaleList();
assertNotNull(list);
assertSame(list, list2);
assertUnmodifiableCollection(list);
Locale[] jdkLocaleArray = Locale.getAvailableLocales();
List jdkLocaleList = Arrays.asList(jdkLocaleArray);
assertEquals(jdkLocaleList, list);
}
//-----------------------------------------------------------------------
/**
* Test availableLocaleSet() method.
*/
public void testAvailableLocaleSet() {
Set set = LocaleUtils.availableLocaleSet();
Set set2 = LocaleUtils.availableLocaleSet();
assertNotNull(set);
assertSame(set, set2);
assertUnmodifiableCollection(set);
Locale[] jdkLocaleArray = Locale.getAvailableLocales();
List jdkLocaleList = Arrays.asList(jdkLocaleArray);
Set jdkLocaleSet = new HashSet(jdkLocaleList);
assertEquals(jdkLocaleSet, set);
}
//-----------------------------------------------------------------------
/**
* Test availableLocaleSet() method.
*/
public void testIsAvailableLocale() {
Set set = LocaleUtils.availableLocaleSet();
assertEquals(set.contains(LOCALE_EN), LocaleUtils.isAvailableLocale(LOCALE_EN));
assertEquals(set.contains(LOCALE_EN_US), LocaleUtils.isAvailableLocale(LOCALE_EN_US));
assertEquals(set.contains(LOCALE_EN_US_ZZZZ), LocaleUtils.isAvailableLocale(LOCALE_EN_US_ZZZZ));
assertEquals(set.contains(LOCALE_FR), LocaleUtils.isAvailableLocale(LOCALE_FR));
assertEquals(set.contains(LOCALE_FR_CA), LocaleUtils.isAvailableLocale(LOCALE_FR_CA));
assertEquals(set.contains(LOCALE_QQ), LocaleUtils.isAvailableLocale(LOCALE_QQ));
assertEquals(set.contains(LOCALE_QQ_ZZ), LocaleUtils.isAvailableLocale(LOCALE_QQ_ZZ));
}
//-----------------------------------------------------------------------
/**
* Make sure the language by country is correct. It checks that
* the LocaleUtils.languagesByCountry(country) call contains the
* array of languages passed in. It may contain more due to JVM
* variations.
*
* @param country
* @param languages array of languages that should be returned
*/
private void assertLanguageByCountry(String country, String[] languages) {
List list = LocaleUtils.languagesByCountry(country);
List list2 = LocaleUtils.languagesByCountry(country);
assertNotNull(list);
assertSame(list, list2);
//search through langauges
for (int i = 0; i < languages.length; i++) {
Iterator iterator = list.iterator();
boolean found = false;
// see if it was returned by the set
while (iterator.hasNext()) {
Locale locale = (Locale) iterator.next();
// should have an en empty variant
assertTrue(locale.getVariant() == null
|| locale.getVariant().length() == 0);
assertEquals(country, locale.getCountry());
if (languages[i].equals(locale.getLanguage())) {
found = true;
break;
}
}
if (!found) {
fail("Cound not find language: " + languages[i]
+ " for country: " + country);
}
}
assertUnmodifiableCollection(list);
}
/**
* Test languagesByCountry() method.
*/
public void testLanguagesByCountry() {
assertLanguageByCountry(null, new String[0]);
assertLanguageByCountry("GB", new String[]{"en"});
assertLanguageByCountry("ZZ", new String[0]);
assertLanguageByCountry("CH", new String[]{"fr", "de", "it"});
}
//-----------------------------------------------------------------------
/**
* Make sure the country by language is correct. It checks that
* the LocaleUtils.countryByLanguage(language) call contains the
* array of countries passed in. It may contain more due to JVM
* variations.
*
*
* @param language
* @param countries array of countries that should be returned
*/
private void assertCountriesByLanguage(String language, String[] countries) {
List list = LocaleUtils.countriesByLanguage(language);
List list2 = LocaleUtils.countriesByLanguage(language);
assertNotNull(list);
assertSame(list, list2);
//search through langauges
for (int i = 0; i < countries.length; i++) {
Iterator iterator = list.iterator();
boolean found = false;
// see if it was returned by the set
while (iterator.hasNext()) {
Locale locale = (Locale) iterator.next();
// should have an en empty variant
assertTrue(locale.getVariant() == null
|| locale.getVariant().length() == 0);
assertEquals(language, locale.getLanguage());
if (countries[i].equals(locale.getCountry())) {
found = true;
break;
}
}
if (!found) {
fail("Cound not find language: " + countries[i]
+ " for country: " + language);
}
}
assertUnmodifiableCollection(list);
}
/**
* Test countriesByLanguage() method.
*/
public void testCountriesByLanguage() {
assertCountriesByLanguage(null, new String[0]);
assertCountriesByLanguage("de", new String[]{"DE", "CH", "AT", "LU"});
assertCountriesByLanguage("zz", new String[0]);
assertCountriesByLanguage("it", new String[]{"IT", "CH"});
}
/**
* @param coll the collection to check
*/
private static void assertUnmodifiableCollection(Collection coll) {
try {
coll.add("Unmodifiable");
fail();
} catch (UnsupportedOperationException ex) {}
}
/**
* Tests #LANG-328 - only language+variant
*/
public void testLang328() {
assertValidToLocale("fr__POSIX", "fr", "", "POSIX");
}
} | // You are a professional Java test case writer, please create a test case named `testLang328` for the issue `Lang-LANG-328`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Lang-LANG-328
//
// ## Issue-Title:
// LocaleUtils.toLocale() rejects strings with only language+variant
//
// ## Issue-Description:
//
// LocaleUtils.toLocale() throws an exception on strings containing a language and a variant but no country code. For example : fr\_\_POSIX
//
//
// This string can be produced with the JDK by instanciating a Locale with an empty string for the country : new Locale("fr", "", "POSIX").toString(). According to the javadoc for the Locale class a variant is allowed with just a language code or just a country code.
//
//
// Commons Configuration handles this case in its PropertyConverter.toLocale() method. I'd like to replace our implementation by the one provided by LocaleUtils, but our tests fail due to this case.
//
//
//
//
//
public void testLang328() {
| 505 | /**
* Tests #LANG-328 - only language+variant
*/ | 54 | 503 | src/test/org/apache/commons/lang/LocaleUtilsTest.java | src/test | ```markdown
## Issue-ID: Lang-LANG-328
## Issue-Title:
LocaleUtils.toLocale() rejects strings with only language+variant
## Issue-Description:
LocaleUtils.toLocale() throws an exception on strings containing a language and a variant but no country code. For example : fr\_\_POSIX
This string can be produced with the JDK by instanciating a Locale with an empty string for the country : new Locale("fr", "", "POSIX").toString(). According to the javadoc for the Locale class a variant is allowed with just a language code or just a country code.
Commons Configuration handles this case in its PropertyConverter.toLocale() method. I'd like to replace our implementation by the one provided by LocaleUtils, but our tests fail due to this case.
```
You are a professional Java test case writer, please create a test case named `testLang328` for the issue `Lang-LANG-328`, utilizing the provided issue report information and the following function signature.
```java
public void testLang328() {
```
| 503 | [
"org.apache.commons.lang.LocaleUtils"
] | 75512a7223215d47f431aeab8f88a694e1bf1111eb04615aeb62aad80742abdc | public void testLang328() | // You are a professional Java test case writer, please create a test case named `testLang328` for the issue `Lang-LANG-328`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Lang-LANG-328
//
// ## Issue-Title:
// LocaleUtils.toLocale() rejects strings with only language+variant
//
// ## Issue-Description:
//
// LocaleUtils.toLocale() throws an exception on strings containing a language and a variant but no country code. For example : fr\_\_POSIX
//
//
// This string can be produced with the JDK by instanciating a Locale with an empty string for the country : new Locale("fr", "", "POSIX").toString(). According to the javadoc for the Locale class a variant is allowed with just a language code or just a country code.
//
//
// Commons Configuration handles this case in its PropertyConverter.toLocale() method. I'd like to replace our implementation by the one provided by LocaleUtils, but our tests fail due to this case.
//
//
//
//
//
| Lang | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.lang;
import java.lang.reflect.Constructor;
import java.lang.reflect.Modifier;
import java.util.Arrays;
import java.util.Collection;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Locale;
import java.util.Set;
import junit.framework.Test;
import junit.framework.TestCase;
import junit.framework.TestSuite;
import junit.textui.TestRunner;
/**
* Unit tests for {@link LocaleUtils}.
*
* @author Chris Hyzer
* @author Stephen Colebourne
* @version $Id$
*/
public class LocaleUtilsTest extends TestCase {
private static final Locale LOCALE_EN = new Locale("en", "");
private static final Locale LOCALE_EN_US = new Locale("en", "US");
private static final Locale LOCALE_EN_US_ZZZZ = new Locale("en", "US", "ZZZZ");
private static final Locale LOCALE_FR = new Locale("fr", "");
private static final Locale LOCALE_FR_CA = new Locale("fr", "CA");
private static final Locale LOCALE_QQ = new Locale("qq", "");
private static final Locale LOCALE_QQ_ZZ = new Locale("qq", "ZZ");
/**
* Constructor.
*
* @param name
*/
public LocaleUtilsTest(String name) {
super(name);
}
/**
* Main.
* @param args
*/
public static void main(String[] args) {
TestRunner.run(suite());
}
/**
* Run the test cases as a suite.
* @return the Test
*/
public static Test suite() {
TestSuite suite = new TestSuite(LocaleUtilsTest.class);
suite.setName("LocaleUtilsTest Tests");
return suite;
}
public void setUp() throws Exception {
super.setUp();
// Testing #LANG-304. Must be called before availableLocaleSet is called.
LocaleUtils.isAvailableLocale(Locale.getDefault());
}
//-----------------------------------------------------------------------
/**
* Test that constructors are public, and work, etc.
*/
public void testConstructor() {
assertNotNull(new LocaleUtils());
Constructor[] cons = LocaleUtils.class.getDeclaredConstructors();
assertEquals(1, cons.length);
assertEquals(true, Modifier.isPublic(cons[0].getModifiers()));
assertEquals(true, Modifier.isPublic(LocaleUtils.class.getModifiers()));
assertEquals(false, Modifier.isFinal(LocaleUtils.class.getModifiers()));
}
//-----------------------------------------------------------------------
/**
* Pass in a valid language, test toLocale.
*
* @param language the language string
*/
private void assertValidToLocale(String language) {
Locale locale = LocaleUtils.toLocale(language);
assertNotNull("valid locale", locale);
assertEquals(language, locale.getLanguage());
//country and variant are empty
assertTrue(locale.getCountry() == null || locale.getCountry().length() == 0);
assertTrue(locale.getVariant() == null || locale.getVariant().length() == 0);
}
/**
* Pass in a valid language, test toLocale.
*
* @param localeString to pass to toLocale()
* @param language of the resulting Locale
* @param country of the resulting Locale
*/
private void assertValidToLocale(String localeString, String language, String country) {
Locale locale = LocaleUtils.toLocale(localeString);
assertNotNull("valid locale", locale);
assertEquals(language, locale.getLanguage());
assertEquals(country, locale.getCountry());
//variant is empty
assertTrue(locale.getVariant() == null || locale.getVariant().length() == 0);
}
/**
* Pass in a valid language, test toLocale.
*
* @param localeString to pass to toLocale()
* @param language of the resulting Locale
* @param country of the resulting Locale
* @param variant of the resulting Locale
*/
private void assertValidToLocale(
String localeString, String language,
String country, String variant) {
Locale locale = LocaleUtils.toLocale(localeString);
assertNotNull("valid locale", locale);
assertEquals(language, locale.getLanguage());
assertEquals(country, locale.getCountry());
assertEquals(variant, locale.getVariant());
}
/**
* Test toLocale() method.
*/
public void testToLocale_1Part() {
assertEquals(null, LocaleUtils.toLocale((String) null));
assertValidToLocale("us");
assertValidToLocale("fr");
assertValidToLocale("de");
assertValidToLocale("zh");
// Valid format but lang doesnt exist, should make instance anyway
assertValidToLocale("qq");
try {
LocaleUtils.toLocale("Us");
fail("Should fail if not lowercase");
} catch (IllegalArgumentException iae) {}
try {
LocaleUtils.toLocale("US");
fail("Should fail if not lowercase");
} catch (IllegalArgumentException iae) {}
try {
LocaleUtils.toLocale("uS");
fail("Should fail if not lowercase");
} catch (IllegalArgumentException iae) {}
try {
LocaleUtils.toLocale("u#");
fail("Should fail if not lowercase");
} catch (IllegalArgumentException iae) {}
try {
LocaleUtils.toLocale("u");
fail("Must be 2 chars if less than 5");
} catch (IllegalArgumentException iae) {}
try {
LocaleUtils.toLocale("uuu");
fail("Must be 2 chars if less than 5");
} catch (IllegalArgumentException iae) {}
try {
LocaleUtils.toLocale("uu_U");
fail("Must be 2 chars if less than 5");
} catch (IllegalArgumentException iae) {}
}
/**
* Test toLocale() method.
*/
public void testToLocale_2Part() {
assertValidToLocale("us_EN", "us", "EN");
//valid though doesnt exist
assertValidToLocale("us_ZH", "us", "ZH");
try {
LocaleUtils.toLocale("us-EN");
fail("Should fail as not underscore");
} catch (IllegalArgumentException iae) {}
try {
LocaleUtils.toLocale("us_En");
fail("Should fail second part not uppercase");
} catch (IllegalArgumentException iae) {}
try {
LocaleUtils.toLocale("us_en");
fail("Should fail second part not uppercase");
} catch (IllegalArgumentException iae) {}
try {
LocaleUtils.toLocale("us_eN");
fail("Should fail second part not uppercase");
} catch (IllegalArgumentException iae) {}
try {
LocaleUtils.toLocale("uS_EN");
fail("Should fail first part not lowercase");
} catch (IllegalArgumentException iae) {}
try {
LocaleUtils.toLocale("us_E3");
fail("Should fail second part not uppercase");
} catch (IllegalArgumentException iae) {}
}
/**
* Test toLocale() method.
*/
public void testToLocale_3Part() {
assertValidToLocale("us_EN_A", "us", "EN", "A");
// this isn't pretty, but was caused by a jdk bug it seems
// http://bugs.sun.com/bugdatabase/view_bug.do?bug_id=4210525
if (SystemUtils.isJavaVersionAtLeast(1.4f)) {
assertValidToLocale("us_EN_a", "us", "EN", "a");
assertValidToLocale("us_EN_SFsafdFDsdfF", "us", "EN", "SFsafdFDsdfF");
} else {
assertValidToLocale("us_EN_a", "us", "EN", "A");
assertValidToLocale("us_EN_SFsafdFDsdfF", "us", "EN", "SFSAFDFDSDFF");
}
try {
LocaleUtils.toLocale("us_EN-a");
fail("Should fail as not underscore");
} catch (IllegalArgumentException iae) {}
try {
LocaleUtils.toLocale("uu_UU_");
fail("Must be 3, 5 or 7+ in length");
} catch (IllegalArgumentException iae) {}
}
//-----------------------------------------------------------------------
/**
* Helper method for local lookups.
*
* @param locale the input locale
* @param defaultLocale the input default locale
* @param expected expected results
*/
private void assertLocaleLookupList(Locale locale, Locale defaultLocale, Locale[] expected) {
List localeList = defaultLocale == null ?
LocaleUtils.localeLookupList(locale) :
LocaleUtils.localeLookupList(locale, defaultLocale);
assertEquals(expected.length, localeList.size());
assertEquals(Arrays.asList(expected), localeList);
assertUnmodifiableCollection(localeList);
}
//-----------------------------------------------------------------------
/**
* Test localeLookupList() method.
*/
public void testLocaleLookupList_Locale() {
assertLocaleLookupList(null, null, new Locale[0]);
assertLocaleLookupList(LOCALE_QQ, null, new Locale[]{LOCALE_QQ});
assertLocaleLookupList(LOCALE_EN, null, new Locale[]{LOCALE_EN});
assertLocaleLookupList(LOCALE_EN, null, new Locale[]{LOCALE_EN});
assertLocaleLookupList(LOCALE_EN_US, null,
new Locale[] {
LOCALE_EN_US,
LOCALE_EN});
assertLocaleLookupList(LOCALE_EN_US_ZZZZ, null,
new Locale[] {
LOCALE_EN_US_ZZZZ,
LOCALE_EN_US,
LOCALE_EN});
}
/**
* Test localeLookupList() method.
*/
public void testLocaleLookupList_LocaleLocale() {
assertLocaleLookupList(LOCALE_QQ, LOCALE_QQ,
new Locale[]{LOCALE_QQ});
assertLocaleLookupList(LOCALE_EN, LOCALE_EN,
new Locale[]{LOCALE_EN});
assertLocaleLookupList(LOCALE_EN_US, LOCALE_EN_US,
new Locale[]{
LOCALE_EN_US,
LOCALE_EN});
assertLocaleLookupList(LOCALE_EN_US, LOCALE_QQ,
new Locale[] {
LOCALE_EN_US,
LOCALE_EN,
LOCALE_QQ});
assertLocaleLookupList(LOCALE_EN_US, LOCALE_QQ_ZZ,
new Locale[] {
LOCALE_EN_US,
LOCALE_EN,
LOCALE_QQ_ZZ});
assertLocaleLookupList(LOCALE_EN_US_ZZZZ, null,
new Locale[] {
LOCALE_EN_US_ZZZZ,
LOCALE_EN_US,
LOCALE_EN});
assertLocaleLookupList(LOCALE_EN_US_ZZZZ, LOCALE_EN_US_ZZZZ,
new Locale[] {
LOCALE_EN_US_ZZZZ,
LOCALE_EN_US,
LOCALE_EN});
assertLocaleLookupList(LOCALE_EN_US_ZZZZ, LOCALE_QQ,
new Locale[] {
LOCALE_EN_US_ZZZZ,
LOCALE_EN_US,
LOCALE_EN,
LOCALE_QQ});
assertLocaleLookupList(LOCALE_EN_US_ZZZZ, LOCALE_QQ_ZZ,
new Locale[] {
LOCALE_EN_US_ZZZZ,
LOCALE_EN_US,
LOCALE_EN,
LOCALE_QQ_ZZ});
assertLocaleLookupList(LOCALE_FR_CA, LOCALE_EN,
new Locale[] {
LOCALE_FR_CA,
LOCALE_FR,
LOCALE_EN});
}
//-----------------------------------------------------------------------
/**
* Test availableLocaleList() method.
*/
public void testAvailableLocaleList() {
List list = LocaleUtils.availableLocaleList();
List list2 = LocaleUtils.availableLocaleList();
assertNotNull(list);
assertSame(list, list2);
assertUnmodifiableCollection(list);
Locale[] jdkLocaleArray = Locale.getAvailableLocales();
List jdkLocaleList = Arrays.asList(jdkLocaleArray);
assertEquals(jdkLocaleList, list);
}
//-----------------------------------------------------------------------
/**
* Test availableLocaleSet() method.
*/
public void testAvailableLocaleSet() {
Set set = LocaleUtils.availableLocaleSet();
Set set2 = LocaleUtils.availableLocaleSet();
assertNotNull(set);
assertSame(set, set2);
assertUnmodifiableCollection(set);
Locale[] jdkLocaleArray = Locale.getAvailableLocales();
List jdkLocaleList = Arrays.asList(jdkLocaleArray);
Set jdkLocaleSet = new HashSet(jdkLocaleList);
assertEquals(jdkLocaleSet, set);
}
//-----------------------------------------------------------------------
/**
* Test availableLocaleSet() method.
*/
public void testIsAvailableLocale() {
Set set = LocaleUtils.availableLocaleSet();
assertEquals(set.contains(LOCALE_EN), LocaleUtils.isAvailableLocale(LOCALE_EN));
assertEquals(set.contains(LOCALE_EN_US), LocaleUtils.isAvailableLocale(LOCALE_EN_US));
assertEquals(set.contains(LOCALE_EN_US_ZZZZ), LocaleUtils.isAvailableLocale(LOCALE_EN_US_ZZZZ));
assertEquals(set.contains(LOCALE_FR), LocaleUtils.isAvailableLocale(LOCALE_FR));
assertEquals(set.contains(LOCALE_FR_CA), LocaleUtils.isAvailableLocale(LOCALE_FR_CA));
assertEquals(set.contains(LOCALE_QQ), LocaleUtils.isAvailableLocale(LOCALE_QQ));
assertEquals(set.contains(LOCALE_QQ_ZZ), LocaleUtils.isAvailableLocale(LOCALE_QQ_ZZ));
}
//-----------------------------------------------------------------------
/**
* Make sure the language by country is correct. It checks that
* the LocaleUtils.languagesByCountry(country) call contains the
* array of languages passed in. It may contain more due to JVM
* variations.
*
* @param country
* @param languages array of languages that should be returned
*/
private void assertLanguageByCountry(String country, String[] languages) {
List list = LocaleUtils.languagesByCountry(country);
List list2 = LocaleUtils.languagesByCountry(country);
assertNotNull(list);
assertSame(list, list2);
//search through langauges
for (int i = 0; i < languages.length; i++) {
Iterator iterator = list.iterator();
boolean found = false;
// see if it was returned by the set
while (iterator.hasNext()) {
Locale locale = (Locale) iterator.next();
// should have an en empty variant
assertTrue(locale.getVariant() == null
|| locale.getVariant().length() == 0);
assertEquals(country, locale.getCountry());
if (languages[i].equals(locale.getLanguage())) {
found = true;
break;
}
}
if (!found) {
fail("Cound not find language: " + languages[i]
+ " for country: " + country);
}
}
assertUnmodifiableCollection(list);
}
/**
* Test languagesByCountry() method.
*/
public void testLanguagesByCountry() {
assertLanguageByCountry(null, new String[0]);
assertLanguageByCountry("GB", new String[]{"en"});
assertLanguageByCountry("ZZ", new String[0]);
assertLanguageByCountry("CH", new String[]{"fr", "de", "it"});
}
//-----------------------------------------------------------------------
/**
* Make sure the country by language is correct. It checks that
* the LocaleUtils.countryByLanguage(language) call contains the
* array of countries passed in. It may contain more due to JVM
* variations.
*
*
* @param language
* @param countries array of countries that should be returned
*/
private void assertCountriesByLanguage(String language, String[] countries) {
List list = LocaleUtils.countriesByLanguage(language);
List list2 = LocaleUtils.countriesByLanguage(language);
assertNotNull(list);
assertSame(list, list2);
//search through langauges
for (int i = 0; i < countries.length; i++) {
Iterator iterator = list.iterator();
boolean found = false;
// see if it was returned by the set
while (iterator.hasNext()) {
Locale locale = (Locale) iterator.next();
// should have an en empty variant
assertTrue(locale.getVariant() == null
|| locale.getVariant().length() == 0);
assertEquals(language, locale.getLanguage());
if (countries[i].equals(locale.getCountry())) {
found = true;
break;
}
}
if (!found) {
fail("Cound not find language: " + countries[i]
+ " for country: " + language);
}
}
assertUnmodifiableCollection(list);
}
/**
* Test countriesByLanguage() method.
*/
public void testCountriesByLanguage() {
assertCountriesByLanguage(null, new String[0]);
assertCountriesByLanguage("de", new String[]{"DE", "CH", "AT", "LU"});
assertCountriesByLanguage("zz", new String[0]);
assertCountriesByLanguage("it", new String[]{"IT", "CH"});
}
/**
* @param coll the collection to check
*/
private static void assertUnmodifiableCollection(Collection coll) {
try {
coll.add("Unmodifiable");
fail();
} catch (UnsupportedOperationException ex) {}
}
/**
* Tests #LANG-328 - only language+variant
*/
public void testLang328() {
assertValidToLocale("fr__POSIX", "fr", "", "POSIX");
}
} |
|
public void testContainsIgnoreCase_LocaleIndependence() {
Locale orig = Locale.getDefault();
Locale[] locales = { Locale.ENGLISH, new Locale("tr"), Locale.getDefault() };
String[][] tdata = {
{ "i", "I" },
{ "I", "i" },
{ "\u03C2", "\u03C3" },
{ "\u03A3", "\u03C2" },
{ "\u03A3", "\u03C3" },
};
String[][] fdata = {
{ "\u00DF", "SS" },
};
try {
for (int i = 0; i < locales.length; i++) {
Locale.setDefault(locales[i]);
for (int j = 0; j < tdata.length; j++) {
assertTrue(Locale.getDefault() + ": " + j + " " + tdata[j][0] + " " + tdata[j][1], StringUtils
.containsIgnoreCase(tdata[j][0], tdata[j][1]));
}
for (int j = 0; j < fdata.length; j++) {
assertFalse(Locale.getDefault() + ": " + j + " " + fdata[j][0] + " " + fdata[j][1], StringUtils
.containsIgnoreCase(fdata[j][0], fdata[j][1]));
}
}
} finally {
Locale.setDefault(orig);
}
} | org.apache.commons.lang.StringUtilsEqualsIndexOfTest::testContainsIgnoreCase_LocaleIndependence | src/test/org/apache/commons/lang/StringUtilsEqualsIndexOfTest.java | 347 | src/test/org/apache/commons/lang/StringUtilsEqualsIndexOfTest.java | testContainsIgnoreCase_LocaleIndependence | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.lang;
import java.util.Locale;
import junit.framework.Test;
import junit.framework.TestCase;
import junit.framework.TestSuite;
import junit.textui.TestRunner;
/**
* Unit tests {@link org.apache.commons.lang.StringUtils} - Substring methods
*
* @author <a href="mailto:[email protected]">Stephen Colebourne</a>
* @author <a href="mailto:[email protected]">Ringo De Smet</a>
* @author Phil Steitz
* @version $Id$
*/
public class StringUtilsEqualsIndexOfTest extends TestCase {
private static final String FOO = "foo";
private static final String BAR = "bar";
private static final String FOOBAR = "foobar";
private static final String[] FOOBAR_SUB_ARRAY = new String[] {"ob", "ba"};
public StringUtilsEqualsIndexOfTest(String name) {
super(name);
}
public static void main(String[] args) {
TestRunner.run(suite());
}
public static Test suite() {
TestSuite suite = new TestSuite(StringUtilsEqualsIndexOfTest.class);
suite.setName("StringUtilsEqualsIndexOf Tests");
return suite;
}
@Override
protected void setUp() throws Exception {
super.setUp();
}
@Override
protected void tearDown() throws Exception {
super.tearDown();
}
//-----------------------------------------------------------------------
public void testEquals() {
assertEquals(true, StringUtils.equals(null, null));
assertEquals(true, StringUtils.equals(FOO, FOO));
assertEquals(true, StringUtils.equals(FOO, new String(new char[] { 'f', 'o', 'o' })));
assertEquals(false, StringUtils.equals(FOO, new String(new char[] { 'f', 'O', 'O' })));
assertEquals(false, StringUtils.equals(FOO, BAR));
assertEquals(false, StringUtils.equals(FOO, null));
assertEquals(false, StringUtils.equals(null, FOO));
}
public void testEqualsIgnoreCase() {
assertEquals(true, StringUtils.equalsIgnoreCase(null, null));
assertEquals(true, StringUtils.equalsIgnoreCase(FOO, FOO));
assertEquals(true, StringUtils.equalsIgnoreCase(FOO, new String(new char[] { 'f', 'o', 'o' })));
assertEquals(true, StringUtils.equalsIgnoreCase(FOO, new String(new char[] { 'f', 'O', 'O' })));
assertEquals(false, StringUtils.equalsIgnoreCase(FOO, BAR));
assertEquals(false, StringUtils.equalsIgnoreCase(FOO, null));
assertEquals(false, StringUtils.equalsIgnoreCase(null, FOO));
}
//-----------------------------------------------------------------------
public void testIndexOf_char() {
assertEquals(-1, StringUtils.indexOf(null, ' '));
assertEquals(-1, StringUtils.indexOf("", ' '));
assertEquals(0, StringUtils.indexOf("aabaabaa", 'a'));
assertEquals(2, StringUtils.indexOf("aabaabaa", 'b'));
}
public void testIndexOf_charInt() {
assertEquals(-1, StringUtils.indexOf(null, ' ', 0));
assertEquals(-1, StringUtils.indexOf(null, ' ', -1));
assertEquals(-1, StringUtils.indexOf("", ' ', 0));
assertEquals(-1, StringUtils.indexOf("", ' ', -1));
assertEquals(0, StringUtils.indexOf("aabaabaa", 'a', 0));
assertEquals(2, StringUtils.indexOf("aabaabaa", 'b', 0));
assertEquals(5, StringUtils.indexOf("aabaabaa", 'b', 3));
assertEquals(-1, StringUtils.indexOf("aabaabaa", 'b', 9));
assertEquals(2, StringUtils.indexOf("aabaabaa", 'b', -1));
}
public void testIndexOf_String() {
assertEquals(-1, StringUtils.indexOf(null, null));
assertEquals(-1, StringUtils.indexOf("", null));
assertEquals(0, StringUtils.indexOf("", ""));
assertEquals(0, StringUtils.indexOf("aabaabaa", "a"));
assertEquals(2, StringUtils.indexOf("aabaabaa", "b"));
assertEquals(1, StringUtils.indexOf("aabaabaa", "ab"));
assertEquals(0, StringUtils.indexOf("aabaabaa", ""));
}
public void testOrdinalIndexOf() {
assertEquals(-1, StringUtils.ordinalIndexOf(null, null, Integer.MIN_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("", null, Integer.MIN_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("", "", Integer.MIN_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "a", Integer.MIN_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "b", Integer.MIN_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "ab", Integer.MIN_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "", Integer.MIN_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf(null, null, -1));
assertEquals(-1, StringUtils.ordinalIndexOf("", null, -1));
assertEquals(-1, StringUtils.ordinalIndexOf("", "", -1));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "a", -1));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "b", -1));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "ab", -1));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "", -1));
assertEquals(-1, StringUtils.ordinalIndexOf(null, null, 0));
assertEquals(-1, StringUtils.ordinalIndexOf("", null, 0));
assertEquals(-1, StringUtils.ordinalIndexOf("", "", 0));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "a", 0));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "b", 0));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "ab", 0));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "", 0));
assertEquals(-1, StringUtils.ordinalIndexOf(null, null, 1));
assertEquals(-1, StringUtils.ordinalIndexOf("", null, 1));
assertEquals(0, StringUtils.ordinalIndexOf("", "", 1));
assertEquals(0, StringUtils.ordinalIndexOf("aabaabaa", "a", 1));
assertEquals(2, StringUtils.ordinalIndexOf("aabaabaa", "b", 1));
assertEquals(1, StringUtils.ordinalIndexOf("aabaabaa", "ab", 1));
assertEquals(0, StringUtils.ordinalIndexOf("aabaabaa", "", 1));
assertEquals(-1, StringUtils.ordinalIndexOf(null, null, 2));
assertEquals(-1, StringUtils.ordinalIndexOf("", null, 2));
assertEquals(0, StringUtils.ordinalIndexOf("", "", 2));
assertEquals(1, StringUtils.ordinalIndexOf("aabaabaa", "a", 2));
assertEquals(5, StringUtils.ordinalIndexOf("aabaabaa", "b", 2));
assertEquals(4, StringUtils.ordinalIndexOf("aabaabaa", "ab", 2));
assertEquals(0, StringUtils.ordinalIndexOf("aabaabaa", "", 2));
assertEquals(-1, StringUtils.ordinalIndexOf(null, null, Integer.MAX_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("", null, Integer.MAX_VALUE));
assertEquals(0, StringUtils.ordinalIndexOf("", "", Integer.MAX_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "a", Integer.MAX_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "b", Integer.MAX_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "ab", Integer.MAX_VALUE));
assertEquals(0, StringUtils.ordinalIndexOf("aabaabaa", "", Integer.MAX_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 0));
assertEquals(0, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 1));
assertEquals(1, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 2));
assertEquals(2, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 3));
assertEquals(3, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 4));
assertEquals(4, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 5));
assertEquals(5, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 6));
assertEquals(6, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 7));
assertEquals(7, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 8));
assertEquals(8, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 9));
assertEquals(-1, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 10));
}
public void testIndexOf_StringInt() {
assertEquals(-1, StringUtils.indexOf(null, null, 0));
assertEquals(-1, StringUtils.indexOf(null, null, -1));
assertEquals(-1, StringUtils.indexOf(null, "", 0));
assertEquals(-1, StringUtils.indexOf(null, "", -1));
assertEquals(-1, StringUtils.indexOf("", null, 0));
assertEquals(-1, StringUtils.indexOf("", null, -1));
assertEquals(0, StringUtils.indexOf("", "", 0));
assertEquals(0, StringUtils.indexOf("", "", -1));
assertEquals(0, StringUtils.indexOf("", "", 9));
assertEquals(0, StringUtils.indexOf("abc", "", 0));
assertEquals(0, StringUtils.indexOf("abc", "", -1));
assertEquals(3, StringUtils.indexOf("abc", "", 9));
assertEquals(3, StringUtils.indexOf("abc", "", 3));
assertEquals(0, StringUtils.indexOf("aabaabaa", "a", 0));
assertEquals(2, StringUtils.indexOf("aabaabaa", "b", 0));
assertEquals(1, StringUtils.indexOf("aabaabaa", "ab", 0));
assertEquals(5, StringUtils.indexOf("aabaabaa", "b", 3));
assertEquals(-1, StringUtils.indexOf("aabaabaa", "b", 9));
assertEquals(2, StringUtils.indexOf("aabaabaa", "b", -1));
assertEquals(2,StringUtils.indexOf("aabaabaa", "", 2));
}
//-----------------------------------------------------------------------
public void testLastIndexOf_char() {
assertEquals(-1, StringUtils.lastIndexOf(null, ' '));
assertEquals(-1, StringUtils.lastIndexOf("", ' '));
assertEquals(7, StringUtils.lastIndexOf("aabaabaa", 'a'));
assertEquals(5, StringUtils.lastIndexOf("aabaabaa", 'b'));
}
public void testLastIndexOf_charInt() {
assertEquals(-1, StringUtils.lastIndexOf(null, ' ', 0));
assertEquals(-1, StringUtils.lastIndexOf(null, ' ', -1));
assertEquals(-1, StringUtils.lastIndexOf("", ' ', 0));
assertEquals(-1, StringUtils.lastIndexOf("", ' ', -1));
assertEquals(7, StringUtils.lastIndexOf("aabaabaa", 'a', 8));
assertEquals(5, StringUtils.lastIndexOf("aabaabaa", 'b', 8));
assertEquals(2, StringUtils.lastIndexOf("aabaabaa", 'b', 3));
assertEquals(5, StringUtils.lastIndexOf("aabaabaa", 'b', 9));
assertEquals(-1, StringUtils.lastIndexOf("aabaabaa", 'b', -1));
assertEquals(0, StringUtils.lastIndexOf("aabaabaa", 'a', 0));
}
public void testLastIndexOf_String() {
assertEquals(-1, StringUtils.lastIndexOf(null, null));
assertEquals(-1, StringUtils.lastIndexOf("", null));
assertEquals(-1, StringUtils.lastIndexOf("", "a"));
assertEquals(0, StringUtils.lastIndexOf("", ""));
assertEquals(8, StringUtils.lastIndexOf("aabaabaa", ""));
assertEquals(7, StringUtils.lastIndexOf("aabaabaa", "a"));
assertEquals(5, StringUtils.lastIndexOf("aabaabaa", "b"));
assertEquals(4, StringUtils.lastIndexOf("aabaabaa", "ab"));
}
public void testLastIndexOf_StringInt() {
assertEquals(-1, StringUtils.lastIndexOf(null, null, 0));
assertEquals(-1, StringUtils.lastIndexOf(null, null, -1));
assertEquals(-1, StringUtils.lastIndexOf(null, "", 0));
assertEquals(-1, StringUtils.lastIndexOf(null, "", -1));
assertEquals(-1, StringUtils.lastIndexOf("", null, 0));
assertEquals(-1, StringUtils.lastIndexOf("", null, -1));
assertEquals(0, StringUtils.lastIndexOf("", "", 0));
assertEquals(-1, StringUtils.lastIndexOf("", "", -1));
assertEquals(0, StringUtils.lastIndexOf("", "", 9));
assertEquals(0, StringUtils.lastIndexOf("abc", "", 0));
assertEquals(-1, StringUtils.lastIndexOf("abc", "", -1));
assertEquals(3, StringUtils.lastIndexOf("abc", "", 9));
assertEquals(7, StringUtils.lastIndexOf("aabaabaa", "a", 8));
assertEquals(5, StringUtils.lastIndexOf("aabaabaa", "b", 8));
assertEquals(4, StringUtils.lastIndexOf("aabaabaa", "ab", 8));
assertEquals(2, StringUtils.lastIndexOf("aabaabaa", "b", 3));
assertEquals(5, StringUtils.lastIndexOf("aabaabaa", "b", 9));
assertEquals(-1, StringUtils.lastIndexOf("aabaabaa", "b", -1));
assertEquals(-1, StringUtils.lastIndexOf("aabaabaa", "b", 0));
assertEquals(0, StringUtils.lastIndexOf("aabaabaa", "a", 0));
}
//-----------------------------------------------------------------------
public void testContainsChar() {
assertEquals(false, StringUtils.contains(null, ' '));
assertEquals(false, StringUtils.contains("", ' '));
assertEquals(false, StringUtils.contains("",null));
assertEquals(false, StringUtils.contains(null,null));
assertEquals(true, StringUtils.contains("abc", 'a'));
assertEquals(true, StringUtils.contains("abc", 'b'));
assertEquals(true, StringUtils.contains("abc", 'c'));
assertEquals(false, StringUtils.contains("abc", 'z'));
}
public void testContainsString() {
assertEquals(false, StringUtils.contains(null, null));
assertEquals(false, StringUtils.contains(null, ""));
assertEquals(false, StringUtils.contains(null, "a"));
assertEquals(false, StringUtils.contains("", null));
assertEquals(true, StringUtils.contains("", ""));
assertEquals(false, StringUtils.contains("", "a"));
assertEquals(true, StringUtils.contains("abc", "a"));
assertEquals(true, StringUtils.contains("abc", "b"));
assertEquals(true, StringUtils.contains("abc", "c"));
assertEquals(true, StringUtils.contains("abc", "abc"));
assertEquals(false, StringUtils.contains("abc", "z"));
}
public void testContainsIgnoreCase_StringString() {
assertFalse(StringUtils.containsIgnoreCase(null, null));
// Null tests
assertFalse(StringUtils.containsIgnoreCase(null, ""));
assertFalse(StringUtils.containsIgnoreCase(null, "a"));
assertFalse(StringUtils.containsIgnoreCase(null, "abc"));
assertFalse(StringUtils.containsIgnoreCase("", null));
assertFalse(StringUtils.containsIgnoreCase("a", null));
assertFalse(StringUtils.containsIgnoreCase("abc", null));
// Match len = 0
assertTrue(StringUtils.containsIgnoreCase("", ""));
assertTrue(StringUtils.containsIgnoreCase("a", ""));
assertTrue(StringUtils.containsIgnoreCase("abc", ""));
// Match len = 1
assertFalse(StringUtils.containsIgnoreCase("", "a"));
assertTrue(StringUtils.containsIgnoreCase("a", "a"));
assertTrue(StringUtils.containsIgnoreCase("abc", "a"));
assertFalse(StringUtils.containsIgnoreCase("", "A"));
assertTrue(StringUtils.containsIgnoreCase("a", "A"));
assertTrue(StringUtils.containsIgnoreCase("abc", "A"));
// Match len > 1
assertFalse(StringUtils.containsIgnoreCase("", "abc"));
assertFalse(StringUtils.containsIgnoreCase("a", "abc"));
assertTrue(StringUtils.containsIgnoreCase("xabcz", "abc"));
assertFalse(StringUtils.containsIgnoreCase("", "ABC"));
assertFalse(StringUtils.containsIgnoreCase("a", "ABC"));
assertTrue(StringUtils.containsIgnoreCase("xabcz", "ABC"));
}
public void testContainsIgnoreCase_LocaleIndependence() {
Locale orig = Locale.getDefault();
Locale[] locales = { Locale.ENGLISH, new Locale("tr"), Locale.getDefault() };
String[][] tdata = {
{ "i", "I" },
{ "I", "i" },
{ "\u03C2", "\u03C3" },
{ "\u03A3", "\u03C2" },
{ "\u03A3", "\u03C3" },
};
String[][] fdata = {
{ "\u00DF", "SS" },
};
try {
for (int i = 0; i < locales.length; i++) {
Locale.setDefault(locales[i]);
for (int j = 0; j < tdata.length; j++) {
assertTrue(Locale.getDefault() + ": " + j + " " + tdata[j][0] + " " + tdata[j][1], StringUtils
.containsIgnoreCase(tdata[j][0], tdata[j][1]));
}
for (int j = 0; j < fdata.length; j++) {
assertFalse(Locale.getDefault() + ": " + j + " " + fdata[j][0] + " " + fdata[j][1], StringUtils
.containsIgnoreCase(fdata[j][0], fdata[j][1]));
}
}
} finally {
Locale.setDefault(orig);
}
}
// -----------------------------------------------------------------------
public void testIndexOfAny_StringStringarray() {
assertEquals(-1, StringUtils.indexOfAny(null, (String[]) null));
assertEquals(-1, StringUtils.indexOfAny(null, FOOBAR_SUB_ARRAY));
assertEquals(-1, StringUtils.indexOfAny(FOOBAR, (String[]) null));
assertEquals(2, StringUtils.indexOfAny(FOOBAR, FOOBAR_SUB_ARRAY));
assertEquals(-1, StringUtils.indexOfAny(FOOBAR, new String[0]));
assertEquals(-1, StringUtils.indexOfAny(null, new String[0]));
assertEquals(-1, StringUtils.indexOfAny("", new String[0]));
assertEquals(-1, StringUtils.indexOfAny(FOOBAR, new String[] {"llll"}));
assertEquals(0, StringUtils.indexOfAny(FOOBAR, new String[] {""}));
assertEquals(0, StringUtils.indexOfAny("", new String[] {""}));
assertEquals(-1, StringUtils.indexOfAny("", new String[] {"a"}));
assertEquals(-1, StringUtils.indexOfAny("", new String[] {null}));
assertEquals(-1, StringUtils.indexOfAny(FOOBAR, new String[] {null}));
assertEquals(-1, StringUtils.indexOfAny(null, new String[] {null}));
}
public void testLastIndexOfAny_StringStringarray() {
assertEquals(-1, StringUtils.lastIndexOfAny(null, null));
assertEquals(-1, StringUtils.lastIndexOfAny(null, FOOBAR_SUB_ARRAY));
assertEquals(-1, StringUtils.lastIndexOfAny(FOOBAR, null));
assertEquals(3, StringUtils.lastIndexOfAny(FOOBAR, FOOBAR_SUB_ARRAY));
assertEquals(-1, StringUtils.lastIndexOfAny(FOOBAR, new String[0]));
assertEquals(-1, StringUtils.lastIndexOfAny(null, new String[0]));
assertEquals(-1, StringUtils.lastIndexOfAny("", new String[0]));
assertEquals(-1, StringUtils.lastIndexOfAny(FOOBAR, new String[] {"llll"}));
assertEquals(6, StringUtils.lastIndexOfAny(FOOBAR, new String[] {""}));
assertEquals(0, StringUtils.lastIndexOfAny("", new String[] {""}));
assertEquals(-1, StringUtils.lastIndexOfAny("", new String[] {"a"}));
assertEquals(-1, StringUtils.lastIndexOfAny("", new String[] {null}));
assertEquals(-1, StringUtils.lastIndexOfAny(FOOBAR, new String[] {null}));
assertEquals(-1, StringUtils.lastIndexOfAny(null, new String[] {null}));
}
//-----------------------------------------------------------------------
public void testIndexOfAny_StringChararray() {
assertEquals(-1, StringUtils.indexOfAny(null, (char[]) null));
assertEquals(-1, StringUtils.indexOfAny(null, new char[0]));
assertEquals(-1, StringUtils.indexOfAny(null, new char[] {'a','b'}));
assertEquals(-1, StringUtils.indexOfAny("", (char[]) null));
assertEquals(-1, StringUtils.indexOfAny("", new char[0]));
assertEquals(-1, StringUtils.indexOfAny("", new char[] {'a','b'}));
assertEquals(-1, StringUtils.indexOfAny("zzabyycdxx", (char[]) null));
assertEquals(-1, StringUtils.indexOfAny("zzabyycdxx", new char[0]));
assertEquals(0, StringUtils.indexOfAny("zzabyycdxx", new char[] {'z','a'}));
assertEquals(3, StringUtils.indexOfAny("zzabyycdxx", new char[] {'b','y'}));
assertEquals(-1, StringUtils.indexOfAny("ab", new char[] {'z'}));
}
public void testIndexOfAny_StringString() {
assertEquals(-1, StringUtils.indexOfAny(null, (String) null));
assertEquals(-1, StringUtils.indexOfAny(null, ""));
assertEquals(-1, StringUtils.indexOfAny(null, "ab"));
assertEquals(-1, StringUtils.indexOfAny("", (String) null));
assertEquals(-1, StringUtils.indexOfAny("", ""));
assertEquals(-1, StringUtils.indexOfAny("", "ab"));
assertEquals(-1, StringUtils.indexOfAny("zzabyycdxx", (String) null));
assertEquals(-1, StringUtils.indexOfAny("zzabyycdxx", ""));
assertEquals(0, StringUtils.indexOfAny("zzabyycdxx", "za"));
assertEquals(3, StringUtils.indexOfAny("zzabyycdxx", "by"));
assertEquals(-1, StringUtils.indexOfAny("ab", "z"));
}
//-----------------------------------------------------------------------
public void testContainsAny_StringChararray() {
assertFalse(StringUtils.containsAny(null, (char[]) null));
assertFalse(StringUtils.containsAny(null, new char[0]));
assertFalse(StringUtils.containsAny(null, new char[] {'a','b'}));
assertFalse(StringUtils.containsAny("", (char[]) null));
assertFalse(StringUtils.containsAny("", new char[0]));
assertFalse(StringUtils.containsAny("", new char[] {'a','b'}));
assertFalse(StringUtils.containsAny("zzabyycdxx", (char[]) null));
assertFalse(StringUtils.containsAny("zzabyycdxx", new char[0]));
assertTrue(StringUtils.containsAny("zzabyycdxx", new char[] {'z','a'}));
assertTrue(StringUtils.containsAny("zzabyycdxx", new char[] {'b','y'}));
assertFalse(StringUtils.containsAny("ab", new char[] {'z'}));
}
public void testContainsAny_StringString() {
assertFalse(StringUtils.containsAny(null, (String) null));
assertFalse(StringUtils.containsAny(null, ""));
assertFalse(StringUtils.containsAny(null, "ab"));
assertFalse(StringUtils.containsAny("", (String) null));
assertFalse(StringUtils.containsAny("", ""));
assertFalse(StringUtils.containsAny("", "ab"));
assertFalse(StringUtils.containsAny("zzabyycdxx", (String) null));
assertFalse(StringUtils.containsAny("zzabyycdxx", ""));
assertTrue(StringUtils.containsAny("zzabyycdxx", "za"));
assertTrue(StringUtils.containsAny("zzabyycdxx", "by"));
assertFalse(StringUtils.containsAny("ab", "z"));
}
//-----------------------------------------------------------------------
public void testIndexOfAnyBut_StringChararray() {
assertEquals(-1, StringUtils.indexOfAnyBut(null, (char[]) null));
assertEquals(-1, StringUtils.indexOfAnyBut(null, new char[0]));
assertEquals(-1, StringUtils.indexOfAnyBut(null, new char[] {'a','b'}));
assertEquals(-1, StringUtils.indexOfAnyBut("", (char[]) null));
assertEquals(-1, StringUtils.indexOfAnyBut("", new char[0]));
assertEquals(-1, StringUtils.indexOfAnyBut("", new char[] {'a','b'}));
assertEquals(-1, StringUtils.indexOfAnyBut("zzabyycdxx", (char[]) null));
assertEquals(-1, StringUtils.indexOfAnyBut("zzabyycdxx", new char[0]));
assertEquals(3, StringUtils.indexOfAnyBut("zzabyycdxx", new char[] {'z','a'}));
assertEquals(0, StringUtils.indexOfAnyBut("zzabyycdxx", new char[] {'b','y'}));
assertEquals(0, StringUtils.indexOfAnyBut("ab", new char[] {'z'}));
}
public void testIndexOfAnyBut_StringString() {
assertEquals(-1, StringUtils.indexOfAnyBut(null, (String) null));
assertEquals(-1, StringUtils.indexOfAnyBut(null, ""));
assertEquals(-1, StringUtils.indexOfAnyBut(null, "ab"));
assertEquals(-1, StringUtils.indexOfAnyBut("", (String) null));
assertEquals(-1, StringUtils.indexOfAnyBut("", ""));
assertEquals(-1, StringUtils.indexOfAnyBut("", "ab"));
assertEquals(-1, StringUtils.indexOfAnyBut("zzabyycdxx", (String) null));
assertEquals(-1, StringUtils.indexOfAnyBut("zzabyycdxx", ""));
assertEquals(3, StringUtils.indexOfAnyBut("zzabyycdxx", "za"));
assertEquals(0, StringUtils.indexOfAnyBut("zzabyycdxx", "by"));
assertEquals(0, StringUtils.indexOfAnyBut("ab", "z"));
}
//-----------------------------------------------------------------------
public void testContainsOnly_String() {
String str1 = "a";
String str2 = "b";
String str3 = "ab";
String chars1= "b";
String chars2= "a";
String chars3= "ab";
assertEquals(false, StringUtils.containsOnly(null, (String) null));
assertEquals(false, StringUtils.containsOnly("", (String) null));
assertEquals(false, StringUtils.containsOnly(null, ""));
assertEquals(false, StringUtils.containsOnly(str1, ""));
assertEquals(true, StringUtils.containsOnly("", ""));
assertEquals(true, StringUtils.containsOnly("", chars1));
assertEquals(false, StringUtils.containsOnly(str1, chars1));
assertEquals(true, StringUtils.containsOnly(str1, chars2));
assertEquals(true, StringUtils.containsOnly(str1, chars3));
assertEquals(true, StringUtils.containsOnly(str2, chars1));
assertEquals(false, StringUtils.containsOnly(str2, chars2));
assertEquals(true, StringUtils.containsOnly(str2, chars3));
assertEquals(false, StringUtils.containsOnly(str3, chars1));
assertEquals(false, StringUtils.containsOnly(str3, chars2));
assertEquals(true, StringUtils.containsOnly(str3, chars3));
}
public void testContainsOnly_Chararray() {
String str1 = "a";
String str2 = "b";
String str3 = "ab";
char[] chars1= {'b'};
char[] chars2= {'a'};
char[] chars3= {'a', 'b'};
char[] emptyChars = new char[0];
assertEquals(false, StringUtils.containsOnly(null, (char[]) null));
assertEquals(false, StringUtils.containsOnly("", (char[]) null));
assertEquals(false, StringUtils.containsOnly(null, emptyChars));
assertEquals(false, StringUtils.containsOnly(str1, emptyChars));
assertEquals(true, StringUtils.containsOnly("", emptyChars));
assertEquals(true, StringUtils.containsOnly("", chars1));
assertEquals(false, StringUtils.containsOnly(str1, chars1));
assertEquals(true, StringUtils.containsOnly(str1, chars2));
assertEquals(true, StringUtils.containsOnly(str1, chars3));
assertEquals(true, StringUtils.containsOnly(str2, chars1));
assertEquals(false, StringUtils.containsOnly(str2, chars2));
assertEquals(true, StringUtils.containsOnly(str2, chars3));
assertEquals(false, StringUtils.containsOnly(str3, chars1));
assertEquals(false, StringUtils.containsOnly(str3, chars2));
assertEquals(true, StringUtils.containsOnly(str3, chars3));
}
public void testContainsNone_String() {
String str1 = "a";
String str2 = "b";
String str3 = "ab.";
String chars1= "b";
String chars2= ".";
String chars3= "cd";
assertEquals(true, StringUtils.containsNone(null, (String) null));
assertEquals(true, StringUtils.containsNone("", (String) null));
assertEquals(true, StringUtils.containsNone(null, ""));
assertEquals(true, StringUtils.containsNone(str1, ""));
assertEquals(true, StringUtils.containsNone("", ""));
assertEquals(true, StringUtils.containsNone("", chars1));
assertEquals(true, StringUtils.containsNone(str1, chars1));
assertEquals(true, StringUtils.containsNone(str1, chars2));
assertEquals(true, StringUtils.containsNone(str1, chars3));
assertEquals(false, StringUtils.containsNone(str2, chars1));
assertEquals(true, StringUtils.containsNone(str2, chars2));
assertEquals(true, StringUtils.containsNone(str2, chars3));
assertEquals(false, StringUtils.containsNone(str3, chars1));
assertEquals(false, StringUtils.containsNone(str3, chars2));
assertEquals(true, StringUtils.containsNone(str3, chars3));
}
public void testContainsNone_Chararray() {
String str1 = "a";
String str2 = "b";
String str3 = "ab.";
char[] chars1= {'b'};
char[] chars2= {'.'};
char[] chars3= {'c', 'd'};
char[] emptyChars = new char[0];
assertEquals(true, StringUtils.containsNone(null, (char[]) null));
assertEquals(true, StringUtils.containsNone("", (char[]) null));
assertEquals(true, StringUtils.containsNone(null, emptyChars));
assertEquals(true, StringUtils.containsNone(str1, emptyChars));
assertEquals(true, StringUtils.containsNone("", emptyChars));
assertEquals(true, StringUtils.containsNone("", chars1));
assertEquals(true, StringUtils.containsNone(str1, chars1));
assertEquals(true, StringUtils.containsNone(str1, chars2));
assertEquals(true, StringUtils.containsNone(str1, chars3));
assertEquals(false, StringUtils.containsNone(str2, chars1));
assertEquals(true, StringUtils.containsNone(str2, chars2));
assertEquals(true, StringUtils.containsNone(str2, chars3));
assertEquals(false, StringUtils.containsNone(str3, chars1));
assertEquals(false, StringUtils.containsNone(str3, chars2));
assertEquals(true, StringUtils.containsNone(str3, chars3));
}
} | // You are a professional Java test case writer, please create a test case named `testContainsIgnoreCase_LocaleIndependence` for the issue `Lang-LANG-432`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Lang-LANG-432
//
// ## Issue-Title:
// Fix case-insensitive string handling
//
// ## Issue-Description:
//
// String.to\*Case() is locale-sensitive, this is usually not intended for case-insensitive comparisions. Please see [Common Bug #3](http://www.nabble.com/Re%3A-Common-Bugs-p14931921s177.html) for details.
//
//
//
//
//
public void testContainsIgnoreCase_LocaleIndependence() {
| 347 | 40 | 315 | src/test/org/apache/commons/lang/StringUtilsEqualsIndexOfTest.java | src/test | ```markdown
## Issue-ID: Lang-LANG-432
## Issue-Title:
Fix case-insensitive string handling
## Issue-Description:
String.to\*Case() is locale-sensitive, this is usually not intended for case-insensitive comparisions. Please see [Common Bug #3](http://www.nabble.com/Re%3A-Common-Bugs-p14931921s177.html) for details.
```
You are a professional Java test case writer, please create a test case named `testContainsIgnoreCase_LocaleIndependence` for the issue `Lang-LANG-432`, utilizing the provided issue report information and the following function signature.
```java
public void testContainsIgnoreCase_LocaleIndependence() {
```
| 315 | [
"org.apache.commons.lang.StringUtils"
] | 75a14e0c8b6dee707b891af6c0206d2558da56e6d49ce1884a129fea03e08799 | public void testContainsIgnoreCase_LocaleIndependence() | // You are a professional Java test case writer, please create a test case named `testContainsIgnoreCase_LocaleIndependence` for the issue `Lang-LANG-432`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Lang-LANG-432
//
// ## Issue-Title:
// Fix case-insensitive string handling
//
// ## Issue-Description:
//
// String.to\*Case() is locale-sensitive, this is usually not intended for case-insensitive comparisions. Please see [Common Bug #3](http://www.nabble.com/Re%3A-Common-Bugs-p14931921s177.html) for details.
//
//
//
//
//
| Lang | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.lang;
import java.util.Locale;
import junit.framework.Test;
import junit.framework.TestCase;
import junit.framework.TestSuite;
import junit.textui.TestRunner;
/**
* Unit tests {@link org.apache.commons.lang.StringUtils} - Substring methods
*
* @author <a href="mailto:[email protected]">Stephen Colebourne</a>
* @author <a href="mailto:[email protected]">Ringo De Smet</a>
* @author Phil Steitz
* @version $Id$
*/
public class StringUtilsEqualsIndexOfTest extends TestCase {
private static final String FOO = "foo";
private static final String BAR = "bar";
private static final String FOOBAR = "foobar";
private static final String[] FOOBAR_SUB_ARRAY = new String[] {"ob", "ba"};
public StringUtilsEqualsIndexOfTest(String name) {
super(name);
}
public static void main(String[] args) {
TestRunner.run(suite());
}
public static Test suite() {
TestSuite suite = new TestSuite(StringUtilsEqualsIndexOfTest.class);
suite.setName("StringUtilsEqualsIndexOf Tests");
return suite;
}
@Override
protected void setUp() throws Exception {
super.setUp();
}
@Override
protected void tearDown() throws Exception {
super.tearDown();
}
//-----------------------------------------------------------------------
public void testEquals() {
assertEquals(true, StringUtils.equals(null, null));
assertEquals(true, StringUtils.equals(FOO, FOO));
assertEquals(true, StringUtils.equals(FOO, new String(new char[] { 'f', 'o', 'o' })));
assertEquals(false, StringUtils.equals(FOO, new String(new char[] { 'f', 'O', 'O' })));
assertEquals(false, StringUtils.equals(FOO, BAR));
assertEquals(false, StringUtils.equals(FOO, null));
assertEquals(false, StringUtils.equals(null, FOO));
}
public void testEqualsIgnoreCase() {
assertEquals(true, StringUtils.equalsIgnoreCase(null, null));
assertEquals(true, StringUtils.equalsIgnoreCase(FOO, FOO));
assertEquals(true, StringUtils.equalsIgnoreCase(FOO, new String(new char[] { 'f', 'o', 'o' })));
assertEquals(true, StringUtils.equalsIgnoreCase(FOO, new String(new char[] { 'f', 'O', 'O' })));
assertEquals(false, StringUtils.equalsIgnoreCase(FOO, BAR));
assertEquals(false, StringUtils.equalsIgnoreCase(FOO, null));
assertEquals(false, StringUtils.equalsIgnoreCase(null, FOO));
}
//-----------------------------------------------------------------------
public void testIndexOf_char() {
assertEquals(-1, StringUtils.indexOf(null, ' '));
assertEquals(-1, StringUtils.indexOf("", ' '));
assertEquals(0, StringUtils.indexOf("aabaabaa", 'a'));
assertEquals(2, StringUtils.indexOf("aabaabaa", 'b'));
}
public void testIndexOf_charInt() {
assertEquals(-1, StringUtils.indexOf(null, ' ', 0));
assertEquals(-1, StringUtils.indexOf(null, ' ', -1));
assertEquals(-1, StringUtils.indexOf("", ' ', 0));
assertEquals(-1, StringUtils.indexOf("", ' ', -1));
assertEquals(0, StringUtils.indexOf("aabaabaa", 'a', 0));
assertEquals(2, StringUtils.indexOf("aabaabaa", 'b', 0));
assertEquals(5, StringUtils.indexOf("aabaabaa", 'b', 3));
assertEquals(-1, StringUtils.indexOf("aabaabaa", 'b', 9));
assertEquals(2, StringUtils.indexOf("aabaabaa", 'b', -1));
}
public void testIndexOf_String() {
assertEquals(-1, StringUtils.indexOf(null, null));
assertEquals(-1, StringUtils.indexOf("", null));
assertEquals(0, StringUtils.indexOf("", ""));
assertEquals(0, StringUtils.indexOf("aabaabaa", "a"));
assertEquals(2, StringUtils.indexOf("aabaabaa", "b"));
assertEquals(1, StringUtils.indexOf("aabaabaa", "ab"));
assertEquals(0, StringUtils.indexOf("aabaabaa", ""));
}
public void testOrdinalIndexOf() {
assertEquals(-1, StringUtils.ordinalIndexOf(null, null, Integer.MIN_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("", null, Integer.MIN_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("", "", Integer.MIN_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "a", Integer.MIN_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "b", Integer.MIN_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "ab", Integer.MIN_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "", Integer.MIN_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf(null, null, -1));
assertEquals(-1, StringUtils.ordinalIndexOf("", null, -1));
assertEquals(-1, StringUtils.ordinalIndexOf("", "", -1));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "a", -1));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "b", -1));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "ab", -1));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "", -1));
assertEquals(-1, StringUtils.ordinalIndexOf(null, null, 0));
assertEquals(-1, StringUtils.ordinalIndexOf("", null, 0));
assertEquals(-1, StringUtils.ordinalIndexOf("", "", 0));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "a", 0));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "b", 0));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "ab", 0));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "", 0));
assertEquals(-1, StringUtils.ordinalIndexOf(null, null, 1));
assertEquals(-1, StringUtils.ordinalIndexOf("", null, 1));
assertEquals(0, StringUtils.ordinalIndexOf("", "", 1));
assertEquals(0, StringUtils.ordinalIndexOf("aabaabaa", "a", 1));
assertEquals(2, StringUtils.ordinalIndexOf("aabaabaa", "b", 1));
assertEquals(1, StringUtils.ordinalIndexOf("aabaabaa", "ab", 1));
assertEquals(0, StringUtils.ordinalIndexOf("aabaabaa", "", 1));
assertEquals(-1, StringUtils.ordinalIndexOf(null, null, 2));
assertEquals(-1, StringUtils.ordinalIndexOf("", null, 2));
assertEquals(0, StringUtils.ordinalIndexOf("", "", 2));
assertEquals(1, StringUtils.ordinalIndexOf("aabaabaa", "a", 2));
assertEquals(5, StringUtils.ordinalIndexOf("aabaabaa", "b", 2));
assertEquals(4, StringUtils.ordinalIndexOf("aabaabaa", "ab", 2));
assertEquals(0, StringUtils.ordinalIndexOf("aabaabaa", "", 2));
assertEquals(-1, StringUtils.ordinalIndexOf(null, null, Integer.MAX_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("", null, Integer.MAX_VALUE));
assertEquals(0, StringUtils.ordinalIndexOf("", "", Integer.MAX_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "a", Integer.MAX_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "b", Integer.MAX_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "ab", Integer.MAX_VALUE));
assertEquals(0, StringUtils.ordinalIndexOf("aabaabaa", "", Integer.MAX_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 0));
assertEquals(0, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 1));
assertEquals(1, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 2));
assertEquals(2, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 3));
assertEquals(3, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 4));
assertEquals(4, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 5));
assertEquals(5, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 6));
assertEquals(6, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 7));
assertEquals(7, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 8));
assertEquals(8, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 9));
assertEquals(-1, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 10));
}
public void testIndexOf_StringInt() {
assertEquals(-1, StringUtils.indexOf(null, null, 0));
assertEquals(-1, StringUtils.indexOf(null, null, -1));
assertEquals(-1, StringUtils.indexOf(null, "", 0));
assertEquals(-1, StringUtils.indexOf(null, "", -1));
assertEquals(-1, StringUtils.indexOf("", null, 0));
assertEquals(-1, StringUtils.indexOf("", null, -1));
assertEquals(0, StringUtils.indexOf("", "", 0));
assertEquals(0, StringUtils.indexOf("", "", -1));
assertEquals(0, StringUtils.indexOf("", "", 9));
assertEquals(0, StringUtils.indexOf("abc", "", 0));
assertEquals(0, StringUtils.indexOf("abc", "", -1));
assertEquals(3, StringUtils.indexOf("abc", "", 9));
assertEquals(3, StringUtils.indexOf("abc", "", 3));
assertEquals(0, StringUtils.indexOf("aabaabaa", "a", 0));
assertEquals(2, StringUtils.indexOf("aabaabaa", "b", 0));
assertEquals(1, StringUtils.indexOf("aabaabaa", "ab", 0));
assertEquals(5, StringUtils.indexOf("aabaabaa", "b", 3));
assertEquals(-1, StringUtils.indexOf("aabaabaa", "b", 9));
assertEquals(2, StringUtils.indexOf("aabaabaa", "b", -1));
assertEquals(2,StringUtils.indexOf("aabaabaa", "", 2));
}
//-----------------------------------------------------------------------
public void testLastIndexOf_char() {
assertEquals(-1, StringUtils.lastIndexOf(null, ' '));
assertEquals(-1, StringUtils.lastIndexOf("", ' '));
assertEquals(7, StringUtils.lastIndexOf("aabaabaa", 'a'));
assertEquals(5, StringUtils.lastIndexOf("aabaabaa", 'b'));
}
public void testLastIndexOf_charInt() {
assertEquals(-1, StringUtils.lastIndexOf(null, ' ', 0));
assertEquals(-1, StringUtils.lastIndexOf(null, ' ', -1));
assertEquals(-1, StringUtils.lastIndexOf("", ' ', 0));
assertEquals(-1, StringUtils.lastIndexOf("", ' ', -1));
assertEquals(7, StringUtils.lastIndexOf("aabaabaa", 'a', 8));
assertEquals(5, StringUtils.lastIndexOf("aabaabaa", 'b', 8));
assertEquals(2, StringUtils.lastIndexOf("aabaabaa", 'b', 3));
assertEquals(5, StringUtils.lastIndexOf("aabaabaa", 'b', 9));
assertEquals(-1, StringUtils.lastIndexOf("aabaabaa", 'b', -1));
assertEquals(0, StringUtils.lastIndexOf("aabaabaa", 'a', 0));
}
public void testLastIndexOf_String() {
assertEquals(-1, StringUtils.lastIndexOf(null, null));
assertEquals(-1, StringUtils.lastIndexOf("", null));
assertEquals(-1, StringUtils.lastIndexOf("", "a"));
assertEquals(0, StringUtils.lastIndexOf("", ""));
assertEquals(8, StringUtils.lastIndexOf("aabaabaa", ""));
assertEquals(7, StringUtils.lastIndexOf("aabaabaa", "a"));
assertEquals(5, StringUtils.lastIndexOf("aabaabaa", "b"));
assertEquals(4, StringUtils.lastIndexOf("aabaabaa", "ab"));
}
public void testLastIndexOf_StringInt() {
assertEquals(-1, StringUtils.lastIndexOf(null, null, 0));
assertEquals(-1, StringUtils.lastIndexOf(null, null, -1));
assertEquals(-1, StringUtils.lastIndexOf(null, "", 0));
assertEquals(-1, StringUtils.lastIndexOf(null, "", -1));
assertEquals(-1, StringUtils.lastIndexOf("", null, 0));
assertEquals(-1, StringUtils.lastIndexOf("", null, -1));
assertEquals(0, StringUtils.lastIndexOf("", "", 0));
assertEquals(-1, StringUtils.lastIndexOf("", "", -1));
assertEquals(0, StringUtils.lastIndexOf("", "", 9));
assertEquals(0, StringUtils.lastIndexOf("abc", "", 0));
assertEquals(-1, StringUtils.lastIndexOf("abc", "", -1));
assertEquals(3, StringUtils.lastIndexOf("abc", "", 9));
assertEquals(7, StringUtils.lastIndexOf("aabaabaa", "a", 8));
assertEquals(5, StringUtils.lastIndexOf("aabaabaa", "b", 8));
assertEquals(4, StringUtils.lastIndexOf("aabaabaa", "ab", 8));
assertEquals(2, StringUtils.lastIndexOf("aabaabaa", "b", 3));
assertEquals(5, StringUtils.lastIndexOf("aabaabaa", "b", 9));
assertEquals(-1, StringUtils.lastIndexOf("aabaabaa", "b", -1));
assertEquals(-1, StringUtils.lastIndexOf("aabaabaa", "b", 0));
assertEquals(0, StringUtils.lastIndexOf("aabaabaa", "a", 0));
}
//-----------------------------------------------------------------------
public void testContainsChar() {
assertEquals(false, StringUtils.contains(null, ' '));
assertEquals(false, StringUtils.contains("", ' '));
assertEquals(false, StringUtils.contains("",null));
assertEquals(false, StringUtils.contains(null,null));
assertEquals(true, StringUtils.contains("abc", 'a'));
assertEquals(true, StringUtils.contains("abc", 'b'));
assertEquals(true, StringUtils.contains("abc", 'c'));
assertEquals(false, StringUtils.contains("abc", 'z'));
}
public void testContainsString() {
assertEquals(false, StringUtils.contains(null, null));
assertEquals(false, StringUtils.contains(null, ""));
assertEquals(false, StringUtils.contains(null, "a"));
assertEquals(false, StringUtils.contains("", null));
assertEquals(true, StringUtils.contains("", ""));
assertEquals(false, StringUtils.contains("", "a"));
assertEquals(true, StringUtils.contains("abc", "a"));
assertEquals(true, StringUtils.contains("abc", "b"));
assertEquals(true, StringUtils.contains("abc", "c"));
assertEquals(true, StringUtils.contains("abc", "abc"));
assertEquals(false, StringUtils.contains("abc", "z"));
}
public void testContainsIgnoreCase_StringString() {
assertFalse(StringUtils.containsIgnoreCase(null, null));
// Null tests
assertFalse(StringUtils.containsIgnoreCase(null, ""));
assertFalse(StringUtils.containsIgnoreCase(null, "a"));
assertFalse(StringUtils.containsIgnoreCase(null, "abc"));
assertFalse(StringUtils.containsIgnoreCase("", null));
assertFalse(StringUtils.containsIgnoreCase("a", null));
assertFalse(StringUtils.containsIgnoreCase("abc", null));
// Match len = 0
assertTrue(StringUtils.containsIgnoreCase("", ""));
assertTrue(StringUtils.containsIgnoreCase("a", ""));
assertTrue(StringUtils.containsIgnoreCase("abc", ""));
// Match len = 1
assertFalse(StringUtils.containsIgnoreCase("", "a"));
assertTrue(StringUtils.containsIgnoreCase("a", "a"));
assertTrue(StringUtils.containsIgnoreCase("abc", "a"));
assertFalse(StringUtils.containsIgnoreCase("", "A"));
assertTrue(StringUtils.containsIgnoreCase("a", "A"));
assertTrue(StringUtils.containsIgnoreCase("abc", "A"));
// Match len > 1
assertFalse(StringUtils.containsIgnoreCase("", "abc"));
assertFalse(StringUtils.containsIgnoreCase("a", "abc"));
assertTrue(StringUtils.containsIgnoreCase("xabcz", "abc"));
assertFalse(StringUtils.containsIgnoreCase("", "ABC"));
assertFalse(StringUtils.containsIgnoreCase("a", "ABC"));
assertTrue(StringUtils.containsIgnoreCase("xabcz", "ABC"));
}
public void testContainsIgnoreCase_LocaleIndependence() {
Locale orig = Locale.getDefault();
Locale[] locales = { Locale.ENGLISH, new Locale("tr"), Locale.getDefault() };
String[][] tdata = {
{ "i", "I" },
{ "I", "i" },
{ "\u03C2", "\u03C3" },
{ "\u03A3", "\u03C2" },
{ "\u03A3", "\u03C3" },
};
String[][] fdata = {
{ "\u00DF", "SS" },
};
try {
for (int i = 0; i < locales.length; i++) {
Locale.setDefault(locales[i]);
for (int j = 0; j < tdata.length; j++) {
assertTrue(Locale.getDefault() + ": " + j + " " + tdata[j][0] + " " + tdata[j][1], StringUtils
.containsIgnoreCase(tdata[j][0], tdata[j][1]));
}
for (int j = 0; j < fdata.length; j++) {
assertFalse(Locale.getDefault() + ": " + j + " " + fdata[j][0] + " " + fdata[j][1], StringUtils
.containsIgnoreCase(fdata[j][0], fdata[j][1]));
}
}
} finally {
Locale.setDefault(orig);
}
}
// -----------------------------------------------------------------------
public void testIndexOfAny_StringStringarray() {
assertEquals(-1, StringUtils.indexOfAny(null, (String[]) null));
assertEquals(-1, StringUtils.indexOfAny(null, FOOBAR_SUB_ARRAY));
assertEquals(-1, StringUtils.indexOfAny(FOOBAR, (String[]) null));
assertEquals(2, StringUtils.indexOfAny(FOOBAR, FOOBAR_SUB_ARRAY));
assertEquals(-1, StringUtils.indexOfAny(FOOBAR, new String[0]));
assertEquals(-1, StringUtils.indexOfAny(null, new String[0]));
assertEquals(-1, StringUtils.indexOfAny("", new String[0]));
assertEquals(-1, StringUtils.indexOfAny(FOOBAR, new String[] {"llll"}));
assertEquals(0, StringUtils.indexOfAny(FOOBAR, new String[] {""}));
assertEquals(0, StringUtils.indexOfAny("", new String[] {""}));
assertEquals(-1, StringUtils.indexOfAny("", new String[] {"a"}));
assertEquals(-1, StringUtils.indexOfAny("", new String[] {null}));
assertEquals(-1, StringUtils.indexOfAny(FOOBAR, new String[] {null}));
assertEquals(-1, StringUtils.indexOfAny(null, new String[] {null}));
}
public void testLastIndexOfAny_StringStringarray() {
assertEquals(-1, StringUtils.lastIndexOfAny(null, null));
assertEquals(-1, StringUtils.lastIndexOfAny(null, FOOBAR_SUB_ARRAY));
assertEquals(-1, StringUtils.lastIndexOfAny(FOOBAR, null));
assertEquals(3, StringUtils.lastIndexOfAny(FOOBAR, FOOBAR_SUB_ARRAY));
assertEquals(-1, StringUtils.lastIndexOfAny(FOOBAR, new String[0]));
assertEquals(-1, StringUtils.lastIndexOfAny(null, new String[0]));
assertEquals(-1, StringUtils.lastIndexOfAny("", new String[0]));
assertEquals(-1, StringUtils.lastIndexOfAny(FOOBAR, new String[] {"llll"}));
assertEquals(6, StringUtils.lastIndexOfAny(FOOBAR, new String[] {""}));
assertEquals(0, StringUtils.lastIndexOfAny("", new String[] {""}));
assertEquals(-1, StringUtils.lastIndexOfAny("", new String[] {"a"}));
assertEquals(-1, StringUtils.lastIndexOfAny("", new String[] {null}));
assertEquals(-1, StringUtils.lastIndexOfAny(FOOBAR, new String[] {null}));
assertEquals(-1, StringUtils.lastIndexOfAny(null, new String[] {null}));
}
//-----------------------------------------------------------------------
public void testIndexOfAny_StringChararray() {
assertEquals(-1, StringUtils.indexOfAny(null, (char[]) null));
assertEquals(-1, StringUtils.indexOfAny(null, new char[0]));
assertEquals(-1, StringUtils.indexOfAny(null, new char[] {'a','b'}));
assertEquals(-1, StringUtils.indexOfAny("", (char[]) null));
assertEquals(-1, StringUtils.indexOfAny("", new char[0]));
assertEquals(-1, StringUtils.indexOfAny("", new char[] {'a','b'}));
assertEquals(-1, StringUtils.indexOfAny("zzabyycdxx", (char[]) null));
assertEquals(-1, StringUtils.indexOfAny("zzabyycdxx", new char[0]));
assertEquals(0, StringUtils.indexOfAny("zzabyycdxx", new char[] {'z','a'}));
assertEquals(3, StringUtils.indexOfAny("zzabyycdxx", new char[] {'b','y'}));
assertEquals(-1, StringUtils.indexOfAny("ab", new char[] {'z'}));
}
public void testIndexOfAny_StringString() {
assertEquals(-1, StringUtils.indexOfAny(null, (String) null));
assertEquals(-1, StringUtils.indexOfAny(null, ""));
assertEquals(-1, StringUtils.indexOfAny(null, "ab"));
assertEquals(-1, StringUtils.indexOfAny("", (String) null));
assertEquals(-1, StringUtils.indexOfAny("", ""));
assertEquals(-1, StringUtils.indexOfAny("", "ab"));
assertEquals(-1, StringUtils.indexOfAny("zzabyycdxx", (String) null));
assertEquals(-1, StringUtils.indexOfAny("zzabyycdxx", ""));
assertEquals(0, StringUtils.indexOfAny("zzabyycdxx", "za"));
assertEquals(3, StringUtils.indexOfAny("zzabyycdxx", "by"));
assertEquals(-1, StringUtils.indexOfAny("ab", "z"));
}
//-----------------------------------------------------------------------
public void testContainsAny_StringChararray() {
assertFalse(StringUtils.containsAny(null, (char[]) null));
assertFalse(StringUtils.containsAny(null, new char[0]));
assertFalse(StringUtils.containsAny(null, new char[] {'a','b'}));
assertFalse(StringUtils.containsAny("", (char[]) null));
assertFalse(StringUtils.containsAny("", new char[0]));
assertFalse(StringUtils.containsAny("", new char[] {'a','b'}));
assertFalse(StringUtils.containsAny("zzabyycdxx", (char[]) null));
assertFalse(StringUtils.containsAny("zzabyycdxx", new char[0]));
assertTrue(StringUtils.containsAny("zzabyycdxx", new char[] {'z','a'}));
assertTrue(StringUtils.containsAny("zzabyycdxx", new char[] {'b','y'}));
assertFalse(StringUtils.containsAny("ab", new char[] {'z'}));
}
public void testContainsAny_StringString() {
assertFalse(StringUtils.containsAny(null, (String) null));
assertFalse(StringUtils.containsAny(null, ""));
assertFalse(StringUtils.containsAny(null, "ab"));
assertFalse(StringUtils.containsAny("", (String) null));
assertFalse(StringUtils.containsAny("", ""));
assertFalse(StringUtils.containsAny("", "ab"));
assertFalse(StringUtils.containsAny("zzabyycdxx", (String) null));
assertFalse(StringUtils.containsAny("zzabyycdxx", ""));
assertTrue(StringUtils.containsAny("zzabyycdxx", "za"));
assertTrue(StringUtils.containsAny("zzabyycdxx", "by"));
assertFalse(StringUtils.containsAny("ab", "z"));
}
//-----------------------------------------------------------------------
public void testIndexOfAnyBut_StringChararray() {
assertEquals(-1, StringUtils.indexOfAnyBut(null, (char[]) null));
assertEquals(-1, StringUtils.indexOfAnyBut(null, new char[0]));
assertEquals(-1, StringUtils.indexOfAnyBut(null, new char[] {'a','b'}));
assertEquals(-1, StringUtils.indexOfAnyBut("", (char[]) null));
assertEquals(-1, StringUtils.indexOfAnyBut("", new char[0]));
assertEquals(-1, StringUtils.indexOfAnyBut("", new char[] {'a','b'}));
assertEquals(-1, StringUtils.indexOfAnyBut("zzabyycdxx", (char[]) null));
assertEquals(-1, StringUtils.indexOfAnyBut("zzabyycdxx", new char[0]));
assertEquals(3, StringUtils.indexOfAnyBut("zzabyycdxx", new char[] {'z','a'}));
assertEquals(0, StringUtils.indexOfAnyBut("zzabyycdxx", new char[] {'b','y'}));
assertEquals(0, StringUtils.indexOfAnyBut("ab", new char[] {'z'}));
}
public void testIndexOfAnyBut_StringString() {
assertEquals(-1, StringUtils.indexOfAnyBut(null, (String) null));
assertEquals(-1, StringUtils.indexOfAnyBut(null, ""));
assertEquals(-1, StringUtils.indexOfAnyBut(null, "ab"));
assertEquals(-1, StringUtils.indexOfAnyBut("", (String) null));
assertEquals(-1, StringUtils.indexOfAnyBut("", ""));
assertEquals(-1, StringUtils.indexOfAnyBut("", "ab"));
assertEquals(-1, StringUtils.indexOfAnyBut("zzabyycdxx", (String) null));
assertEquals(-1, StringUtils.indexOfAnyBut("zzabyycdxx", ""));
assertEquals(3, StringUtils.indexOfAnyBut("zzabyycdxx", "za"));
assertEquals(0, StringUtils.indexOfAnyBut("zzabyycdxx", "by"));
assertEquals(0, StringUtils.indexOfAnyBut("ab", "z"));
}
//-----------------------------------------------------------------------
public void testContainsOnly_String() {
String str1 = "a";
String str2 = "b";
String str3 = "ab";
String chars1= "b";
String chars2= "a";
String chars3= "ab";
assertEquals(false, StringUtils.containsOnly(null, (String) null));
assertEquals(false, StringUtils.containsOnly("", (String) null));
assertEquals(false, StringUtils.containsOnly(null, ""));
assertEquals(false, StringUtils.containsOnly(str1, ""));
assertEquals(true, StringUtils.containsOnly("", ""));
assertEquals(true, StringUtils.containsOnly("", chars1));
assertEquals(false, StringUtils.containsOnly(str1, chars1));
assertEquals(true, StringUtils.containsOnly(str1, chars2));
assertEquals(true, StringUtils.containsOnly(str1, chars3));
assertEquals(true, StringUtils.containsOnly(str2, chars1));
assertEquals(false, StringUtils.containsOnly(str2, chars2));
assertEquals(true, StringUtils.containsOnly(str2, chars3));
assertEquals(false, StringUtils.containsOnly(str3, chars1));
assertEquals(false, StringUtils.containsOnly(str3, chars2));
assertEquals(true, StringUtils.containsOnly(str3, chars3));
}
public void testContainsOnly_Chararray() {
String str1 = "a";
String str2 = "b";
String str3 = "ab";
char[] chars1= {'b'};
char[] chars2= {'a'};
char[] chars3= {'a', 'b'};
char[] emptyChars = new char[0];
assertEquals(false, StringUtils.containsOnly(null, (char[]) null));
assertEquals(false, StringUtils.containsOnly("", (char[]) null));
assertEquals(false, StringUtils.containsOnly(null, emptyChars));
assertEquals(false, StringUtils.containsOnly(str1, emptyChars));
assertEquals(true, StringUtils.containsOnly("", emptyChars));
assertEquals(true, StringUtils.containsOnly("", chars1));
assertEquals(false, StringUtils.containsOnly(str1, chars1));
assertEquals(true, StringUtils.containsOnly(str1, chars2));
assertEquals(true, StringUtils.containsOnly(str1, chars3));
assertEquals(true, StringUtils.containsOnly(str2, chars1));
assertEquals(false, StringUtils.containsOnly(str2, chars2));
assertEquals(true, StringUtils.containsOnly(str2, chars3));
assertEquals(false, StringUtils.containsOnly(str3, chars1));
assertEquals(false, StringUtils.containsOnly(str3, chars2));
assertEquals(true, StringUtils.containsOnly(str3, chars3));
}
public void testContainsNone_String() {
String str1 = "a";
String str2 = "b";
String str3 = "ab.";
String chars1= "b";
String chars2= ".";
String chars3= "cd";
assertEquals(true, StringUtils.containsNone(null, (String) null));
assertEquals(true, StringUtils.containsNone("", (String) null));
assertEquals(true, StringUtils.containsNone(null, ""));
assertEquals(true, StringUtils.containsNone(str1, ""));
assertEquals(true, StringUtils.containsNone("", ""));
assertEquals(true, StringUtils.containsNone("", chars1));
assertEquals(true, StringUtils.containsNone(str1, chars1));
assertEquals(true, StringUtils.containsNone(str1, chars2));
assertEquals(true, StringUtils.containsNone(str1, chars3));
assertEquals(false, StringUtils.containsNone(str2, chars1));
assertEquals(true, StringUtils.containsNone(str2, chars2));
assertEquals(true, StringUtils.containsNone(str2, chars3));
assertEquals(false, StringUtils.containsNone(str3, chars1));
assertEquals(false, StringUtils.containsNone(str3, chars2));
assertEquals(true, StringUtils.containsNone(str3, chars3));
}
public void testContainsNone_Chararray() {
String str1 = "a";
String str2 = "b";
String str3 = "ab.";
char[] chars1= {'b'};
char[] chars2= {'.'};
char[] chars3= {'c', 'd'};
char[] emptyChars = new char[0];
assertEquals(true, StringUtils.containsNone(null, (char[]) null));
assertEquals(true, StringUtils.containsNone("", (char[]) null));
assertEquals(true, StringUtils.containsNone(null, emptyChars));
assertEquals(true, StringUtils.containsNone(str1, emptyChars));
assertEquals(true, StringUtils.containsNone("", emptyChars));
assertEquals(true, StringUtils.containsNone("", chars1));
assertEquals(true, StringUtils.containsNone(str1, chars1));
assertEquals(true, StringUtils.containsNone(str1, chars2));
assertEquals(true, StringUtils.containsNone(str1, chars3));
assertEquals(false, StringUtils.containsNone(str2, chars1));
assertEquals(true, StringUtils.containsNone(str2, chars2));
assertEquals(true, StringUtils.containsNone(str2, chars3));
assertEquals(false, StringUtils.containsNone(str3, chars1));
assertEquals(false, StringUtils.containsNone(str3, chars2));
assertEquals(true, StringUtils.containsNone(str3, chars3));
}
} |
||
public void testIssue779() {
testNotMissing(
"var a = f(); try { alert(); if (a > 0) return 1; }" +
"finally { a = 5; } return 2;");
} | com.google.javascript.jscomp.CheckMissingReturnTest::testIssue779 | test/com/google/javascript/jscomp/CheckMissingReturnTest.java | 197 | test/com/google/javascript/jscomp/CheckMissingReturnTest.java | testIssue779 | /*
* Copyright 2008 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import com.google.javascript.jscomp.CheckLevel;
/**
* Tests for {@link CheckMissingReturn}.
*
*/
public class CheckMissingReturnTest extends CompilerTestCase {
public CheckMissingReturnTest() {
enableTypeCheck(CheckLevel.OFF);
}
@Override
protected CompilerPass getProcessor(final Compiler compiler) {
return new CombinedCompilerPass(compiler,
new CheckMissingReturn(compiler, CheckLevel.ERROR));
}
public void testMissingReturn() {
// Requires control flow analysis.
testMissing("if (a) { return 1; }");
// Switch statement.
testMissing("switch(1) { case 12: return 5; }");
// Test try catch finally.
testMissing("try { foo() } catch (e) { return 5; } finally { }");
// Nested scope.
testMissing("/** @return {number} */ function f() { var x; }; return 1;");
testMissing("/** @return {number} */ function f() { return 1; };");
}
public void testReturnNotMissing() {
// Empty function body. Ignore this case. The remainder of the functions in
// this test have non-empty bodies.
testNotMissing("");
// Simple cases.
testSame("function f() { var x; }");
testNotMissing("return 1;");
// Returning void and undefined.
testNotMissing("void", "var x;");
testNotMissing("undefined", "var x;");
// Returning a union that includes void or undefined.
testNotMissing("number|undefined", "var x;");
testNotMissing("number|void", "var x;");
testNotMissing("(number,void)", "var x;");
testNotMissing("(number,undefined)", "var x;");
testNotMissing("*", "var x;");
// Test try catch finally.
testNotMissing("try { return foo() } catch (e) { } finally { }");
// Nested function.
testNotMissing(
"/** @return {number} */ function f() { return 1; }; return 1;");
// Strange tests that come up when reviewing closure code.
testNotMissing("try { return 12; } finally { return 62; }");
testNotMissing("try { } finally { return 1; }");
testNotMissing("switch(1) { default: return 1; }");
testNotMissing("switch(g) { case 1: return 1; default: return 2; }");
}
public void testFinallyStatements() {
// The control flow analysis (CFA) treats finally blocks somewhat strangely.
// The CFA might indicate that a finally block implicitly returns. However,
// if entry into the finally block is normally caused by an explicit return
// statement, then a return statement isn't missing:
//
// try {
// return 1;
// } finally {
// // CFA determines implicit return. However, return not missing
// // because of return statement in try block.
// }
//
// Hence extra tests are warranted for various cases involving finally
// blocks.
// Simple finally case.
testNotMissing("try { return 1; } finally { }");
testNotMissing("try { } finally { return 1; }");
testMissing("try { } finally { }");
// Cycles in the CFG within the finally block were causing problems before.
testNotMissing("try { return 1; } finally { while (true) { } }");
testMissing("try { } finally { while (x) { } }");
testMissing("try { } finally { while (x) { if (x) { break; } } }");
testNotMissing(
"try { return 2; } finally { while (x) { if (x) { break; } } }");
// Test various cases with nested try statements.
testMissing("try { } finally { try { } finally { } }");
testNotMissing("try { } finally { try { return 1; } finally { } }");
testNotMissing("try { return 1; } finally { try { } finally { } }");
// Calling a function potentially causes control flow to transfer to finally
// block. However, the function will not return in this case as the
// exception will unwind the stack. Hence this function isn't missing a
// return statement (i.e., the running program will not expect a return
// value from the function if an exception is thrown).
testNotMissing("try { g(); return 1; } finally { }");
// Closures within try ... finally affect missing return statement analysis
// because of the nested scopes. The following tests check for missing
// return statements in the three possible configurations: both scopes
// return; enclosed doesn't return; enclosing doesn't return.
testNotMissing(
"try {" +
" /** @return {number} */ function f() {" +
" try { return 1; }" +
" finally { }" +
" };" +
" return 1;" +
"}" +
"finally { }");
testMissing(
"try {" +
" /** @return {number} */ function f() {" +
" try { }" +
" finally { }" +
" };" +
" return 1;" +
"}" +
"finally { }");
testMissing(
"try {" +
" /** @return {number} */ function f() {" +
" try { return 1; }" +
" finally { }" +
" };" +
"}" +
"finally { }");
}
public void testKnownConditions() {
testNotMissing("if (true) return 1");
testMissing("if (true) {} else {return 1}");
testMissing("if (false) return 1");
testNotMissing("if (false) {} else {return 1}");
testNotMissing("if (1) return 1");
testMissing("if (1) {} else {return 1}");
testMissing("if (0) return 1");
testNotMissing("if (0) {} else {return 1}");
testNotMissing("if (3) return 1");
testMissing("if (3) {} else {return 1}");
}
public void testKnownWhileLoop() {
testNotMissing("while (1) return 1");
testNotMissing("while (1) { if (x) {return 1} else {return 1}}");
testNotMissing("while (0) {} return 1");
// TODO(user): The current algorithm will not detect this case. It is
// still computable in most cases.
testNotMissing("while (1) {} return 0");
testMissing("while (false) return 1");
// Not known.
testMissing("while(x) { return 1 }");
}
public void testMultiConditions() {
testMissing("if (a) { } else { while (1) {return 1} }");
testNotMissing("if (a) { return 1} else { while (1) {return 1} }");
}
public void testIssue779() {
testNotMissing(
"var a = f(); try { alert(); if (a > 0) return 1; }" +
"finally { a = 5; } return 2;");
}
private static String createFunction(String returnType, String body) {
return "/** @return {" + returnType + "} */ function foo() {" + body + "}";
}
private void testMissing(String returnType, String body) {
String js = createFunction(returnType, body);
test(js, js, CheckMissingReturn.MISSING_RETURN_STATEMENT);
}
private void testNotMissing(String returnType, String body) {
testSame(createFunction(returnType, body));
}
/** Creates function with return type {number} */
private void testNotMissing(String body) {
testNotMissing("number", body);
}
/** Creates function with return type {number} */
private void testMissing(String body) {
testMissing("number", body);
}
} | // You are a professional Java test case writer, please create a test case named `testIssue779` for the issue `Closure-779`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-779
//
// ## Issue-Title:
// bogus 'missing return' warning
//
// ## Issue-Description:
// The following sample code compiles with "Missing return statement. Function expected to return boolean." warning:
//
// /\*\*
// \* @return {boolean}
// \*/
// function fb(a)
// {
// try
// {
// alert(a); // Some method, which can throw
// if (a > 0)
// return false;
// }
// finally
// {
// a = 5;
// }
//
// return true;
// }
//
//
public void testIssue779() {
| 197 | 14 | 193 | test/com/google/javascript/jscomp/CheckMissingReturnTest.java | test | ```markdown
## Issue-ID: Closure-779
## Issue-Title:
bogus 'missing return' warning
## Issue-Description:
The following sample code compiles with "Missing return statement. Function expected to return boolean." warning:
/\*\*
\* @return {boolean}
\*/
function fb(a)
{
try
{
alert(a); // Some method, which can throw
if (a > 0)
return false;
}
finally
{
a = 5;
}
return true;
}
```
You are a professional Java test case writer, please create a test case named `testIssue779` for the issue `Closure-779`, utilizing the provided issue report information and the following function signature.
```java
public void testIssue779() {
```
| 193 | [
"com.google.javascript.jscomp.ControlFlowAnalysis"
] | 76b2627c4f07659475184fe2e7d8fee81654de85de0fdddb31c46f022bc131ee | public void testIssue779() | // You are a professional Java test case writer, please create a test case named `testIssue779` for the issue `Closure-779`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-779
//
// ## Issue-Title:
// bogus 'missing return' warning
//
// ## Issue-Description:
// The following sample code compiles with "Missing return statement. Function expected to return boolean." warning:
//
// /\*\*
// \* @return {boolean}
// \*/
// function fb(a)
// {
// try
// {
// alert(a); // Some method, which can throw
// if (a > 0)
// return false;
// }
// finally
// {
// a = 5;
// }
//
// return true;
// }
//
//
| Closure | /*
* Copyright 2008 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import com.google.javascript.jscomp.CheckLevel;
/**
* Tests for {@link CheckMissingReturn}.
*
*/
public class CheckMissingReturnTest extends CompilerTestCase {
public CheckMissingReturnTest() {
enableTypeCheck(CheckLevel.OFF);
}
@Override
protected CompilerPass getProcessor(final Compiler compiler) {
return new CombinedCompilerPass(compiler,
new CheckMissingReturn(compiler, CheckLevel.ERROR));
}
public void testMissingReturn() {
// Requires control flow analysis.
testMissing("if (a) { return 1; }");
// Switch statement.
testMissing("switch(1) { case 12: return 5; }");
// Test try catch finally.
testMissing("try { foo() } catch (e) { return 5; } finally { }");
// Nested scope.
testMissing("/** @return {number} */ function f() { var x; }; return 1;");
testMissing("/** @return {number} */ function f() { return 1; };");
}
public void testReturnNotMissing() {
// Empty function body. Ignore this case. The remainder of the functions in
// this test have non-empty bodies.
testNotMissing("");
// Simple cases.
testSame("function f() { var x; }");
testNotMissing("return 1;");
// Returning void and undefined.
testNotMissing("void", "var x;");
testNotMissing("undefined", "var x;");
// Returning a union that includes void or undefined.
testNotMissing("number|undefined", "var x;");
testNotMissing("number|void", "var x;");
testNotMissing("(number,void)", "var x;");
testNotMissing("(number,undefined)", "var x;");
testNotMissing("*", "var x;");
// Test try catch finally.
testNotMissing("try { return foo() } catch (e) { } finally { }");
// Nested function.
testNotMissing(
"/** @return {number} */ function f() { return 1; }; return 1;");
// Strange tests that come up when reviewing closure code.
testNotMissing("try { return 12; } finally { return 62; }");
testNotMissing("try { } finally { return 1; }");
testNotMissing("switch(1) { default: return 1; }");
testNotMissing("switch(g) { case 1: return 1; default: return 2; }");
}
public void testFinallyStatements() {
// The control flow analysis (CFA) treats finally blocks somewhat strangely.
// The CFA might indicate that a finally block implicitly returns. However,
// if entry into the finally block is normally caused by an explicit return
// statement, then a return statement isn't missing:
//
// try {
// return 1;
// } finally {
// // CFA determines implicit return. However, return not missing
// // because of return statement in try block.
// }
//
// Hence extra tests are warranted for various cases involving finally
// blocks.
// Simple finally case.
testNotMissing("try { return 1; } finally { }");
testNotMissing("try { } finally { return 1; }");
testMissing("try { } finally { }");
// Cycles in the CFG within the finally block were causing problems before.
testNotMissing("try { return 1; } finally { while (true) { } }");
testMissing("try { } finally { while (x) { } }");
testMissing("try { } finally { while (x) { if (x) { break; } } }");
testNotMissing(
"try { return 2; } finally { while (x) { if (x) { break; } } }");
// Test various cases with nested try statements.
testMissing("try { } finally { try { } finally { } }");
testNotMissing("try { } finally { try { return 1; } finally { } }");
testNotMissing("try { return 1; } finally { try { } finally { } }");
// Calling a function potentially causes control flow to transfer to finally
// block. However, the function will not return in this case as the
// exception will unwind the stack. Hence this function isn't missing a
// return statement (i.e., the running program will not expect a return
// value from the function if an exception is thrown).
testNotMissing("try { g(); return 1; } finally { }");
// Closures within try ... finally affect missing return statement analysis
// because of the nested scopes. The following tests check for missing
// return statements in the three possible configurations: both scopes
// return; enclosed doesn't return; enclosing doesn't return.
testNotMissing(
"try {" +
" /** @return {number} */ function f() {" +
" try { return 1; }" +
" finally { }" +
" };" +
" return 1;" +
"}" +
"finally { }");
testMissing(
"try {" +
" /** @return {number} */ function f() {" +
" try { }" +
" finally { }" +
" };" +
" return 1;" +
"}" +
"finally { }");
testMissing(
"try {" +
" /** @return {number} */ function f() {" +
" try { return 1; }" +
" finally { }" +
" };" +
"}" +
"finally { }");
}
public void testKnownConditions() {
testNotMissing("if (true) return 1");
testMissing("if (true) {} else {return 1}");
testMissing("if (false) return 1");
testNotMissing("if (false) {} else {return 1}");
testNotMissing("if (1) return 1");
testMissing("if (1) {} else {return 1}");
testMissing("if (0) return 1");
testNotMissing("if (0) {} else {return 1}");
testNotMissing("if (3) return 1");
testMissing("if (3) {} else {return 1}");
}
public void testKnownWhileLoop() {
testNotMissing("while (1) return 1");
testNotMissing("while (1) { if (x) {return 1} else {return 1}}");
testNotMissing("while (0) {} return 1");
// TODO(user): The current algorithm will not detect this case. It is
// still computable in most cases.
testNotMissing("while (1) {} return 0");
testMissing("while (false) return 1");
// Not known.
testMissing("while(x) { return 1 }");
}
public void testMultiConditions() {
testMissing("if (a) { } else { while (1) {return 1} }");
testNotMissing("if (a) { return 1} else { while (1) {return 1} }");
}
public void testIssue779() {
testNotMissing(
"var a = f(); try { alert(); if (a > 0) return 1; }" +
"finally { a = 5; } return 2;");
}
private static String createFunction(String returnType, String body) {
return "/** @return {" + returnType + "} */ function foo() {" + body + "}";
}
private void testMissing(String returnType, String body) {
String js = createFunction(returnType, body);
test(js, js, CheckMissingReturn.MISSING_RETURN_STATEMENT);
}
private void testNotMissing(String returnType, String body) {
testSame(createFunction(returnType, body));
}
/** Creates function with return type {number} */
private void testNotMissing(String body) {
testNotMissing("number", body);
}
/** Creates function with return type {number} */
private void testMissing(String body) {
testMissing("number", body);
}
} |
||
@Test public void handlesAbsPrefixOnHasAttr() {
// 1: no abs url; 2: has abs url
Document doc = Jsoup.parse("<a id=1 href='/foo'>One</a> <a id=2 href='http://jsoup.org/'>Two</a>");
Element one = doc.select("#1").first();
Element two = doc.select("#2").first();
assertFalse(one.hasAttr("abs:href"));
assertTrue(one.hasAttr("href"));
assertEquals("", one.absUrl("href"));
assertTrue(two.hasAttr("abs:href"));
assertTrue(two.hasAttr("href"));
assertEquals("http://jsoup.org/", two.absUrl("href"));
} | org.jsoup.nodes.NodeTest::handlesAbsPrefixOnHasAttr | src/test/java/org/jsoup/nodes/NodeTest.java | 61 | src/test/java/org/jsoup/nodes/NodeTest.java | handlesAbsPrefixOnHasAttr | package org.jsoup.nodes;
import org.jsoup.Jsoup;
import org.jsoup.TextUtil;
import org.jsoup.parser.Tag;
import org.junit.Test;
import static org.junit.Assert.*;
/**
Tests Nodes
@author Jonathan Hedley, [email protected] */
public class NodeTest {
@Test public void handlesBaseUri() {
Tag tag = Tag.valueOf("a");
Attributes attribs = new Attributes();
attribs.put("relHref", "/foo");
attribs.put("absHref", "http://bar/qux");
Element noBase = new Element(tag, "", attribs);
assertEquals("", noBase.absUrl("relHref")); // with no base, should NOT fallback to href attrib, whatever it is
assertEquals("http://bar/qux", noBase.absUrl("absHref")); // no base but valid attrib, return attrib
Element withBase = new Element(tag, "http://foo/", attribs);
assertEquals("http://foo/foo", withBase.absUrl("relHref")); // construct abs from base + rel
assertEquals("http://bar/qux", withBase.absUrl("absHref")); // href is abs, so returns that
assertEquals("", withBase.absUrl("noval"));
Element dodgyBase = new Element(tag, "wtf://no-such-protocol/", attribs);
assertEquals("http://bar/qux", dodgyBase.absUrl("absHref")); // base fails, but href good, so get that
assertEquals("", dodgyBase.absUrl("relHref")); // base fails, only rel href, so return nothing
}
@Test public void handlesAbsPrefix() {
Document doc = Jsoup.parse("<a href=/foo>Hello</a>", "http://jsoup.org/");
Element a = doc.select("a").first();
assertEquals("/foo", a.attr("href"));
assertEquals("http://jsoup.org/foo", a.attr("abs:href"));
assertTrue(a.hasAttr("abs:href"));
}
@Test public void handlesAbsOnImage() {
Document doc = Jsoup.parse("<p><img src=\"/rez/osi_logo.png\" /></p>", "http://jsoup.org/");
Element img = doc.select("img").first();
assertEquals("http://jsoup.org/rez/osi_logo.png", img.attr("abs:src"));
assertEquals(img.absUrl("src"), img.attr("abs:src"));
}
@Test public void handlesAbsPrefixOnHasAttr() {
// 1: no abs url; 2: has abs url
Document doc = Jsoup.parse("<a id=1 href='/foo'>One</a> <a id=2 href='http://jsoup.org/'>Two</a>");
Element one = doc.select("#1").first();
Element two = doc.select("#2").first();
assertFalse(one.hasAttr("abs:href"));
assertTrue(one.hasAttr("href"));
assertEquals("", one.absUrl("href"));
assertTrue(two.hasAttr("abs:href"));
assertTrue(two.hasAttr("href"));
assertEquals("http://jsoup.org/", two.absUrl("href"));
}
@Test public void literalAbsPrefix() {
// if there is a literal attribute "abs:xxx", don't try and make absolute.
Document doc = Jsoup.parse("<a abs:href='odd'>One</a>");
Element el = doc.select("a").first();
assertTrue(el.hasAttr("abs:href"));
assertEquals("odd", el.attr("abs:href"));
}
/*
Test for an issue with Java's abs URL handler.
*/
@Test public void absHandlesRelativeQuery() {
Document doc = Jsoup.parse("<a href='?foo'>One</a> <a href='bar.html?foo'>Two</a>", "http://jsoup.org/path/file?bar");
Element a1 = doc.select("a").first();
assertEquals("http://jsoup.org/path/file?foo", a1.absUrl("href"));
Element a2 = doc.select("a").get(1);
assertEquals("http://jsoup.org/path/bar.html?foo", a2.absUrl("href"));
}
@Test public void testRemove() {
Document doc = Jsoup.parse("<p>One <span>two</span> three</p>");
Element p = doc.select("p").first();
p.childNode(0).remove();
assertEquals("two three", p.text());
assertEquals("<span>two</span> three", TextUtil.stripNewlines(p.html()));
}
@Test public void testReplace() {
Document doc = Jsoup.parse("<p>One <span>two</span> three</p>");
Element p = doc.select("p").first();
Element insert = doc.createElement("em").text("foo");
p.childNode(1).replaceWith(insert);
assertEquals("One <em>foo</em> three", p.html());
}
@Test public void ownerDocument() {
Document doc = Jsoup.parse("<p>Hello");
Element p = doc.select("p").first();
assertTrue(p.ownerDocument() == doc);
assertTrue(doc.ownerDocument() == doc);
assertNull(doc.parent());
}
@Test public void before() {
Document doc = Jsoup.parse("<p>One <b>two</b> three</p>");
Element newNode = new Element(Tag.valueOf("em"), "");
newNode.appendText("four");
doc.select("b").first().before(newNode);
assertEquals("<p>One <em>four</em><b>two</b> three</p>", doc.body().html());
doc.select("b").first().before("<i>five</i>");
assertEquals("<p>One <em>four</em><i>five</i><b>two</b> three</p>", doc.body().html());
}
@Test public void after() {
Document doc = Jsoup.parse("<p>One <b>two</b> three</p>");
Element newNode = new Element(Tag.valueOf("em"), "");
newNode.appendText("four");
doc.select("b").first().after(newNode);
assertEquals("<p>One <b>two</b><em>four</em> three</p>", doc.body().html());
doc.select("b").first().after("<i>five</i>");
assertEquals("<p>One <b>two</b><i>five</i><em>four</em> three</p>", doc.body().html());
}
} | // You are a professional Java test case writer, please create a test case named `handlesAbsPrefixOnHasAttr` for the issue `Jsoup-97`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Jsoup-97
//
// ## Issue-Title:
// abs: attribute prefix does not work on Elements.attr()
//
// ## Issue-Description:
// Elements.attr() iterates on its element to look for the first one with the given attrbute.
//
//
// If I try to get the attribute abs:href, the test element.hasAttr("abs:herf") fails, and the returned value is an empty string.
//
//
//
//
@Test public void handlesAbsPrefixOnHasAttr() {
| 61 | 13 | 48 | src/test/java/org/jsoup/nodes/NodeTest.java | src/test/java | ```markdown
## Issue-ID: Jsoup-97
## Issue-Title:
abs: attribute prefix does not work on Elements.attr()
## Issue-Description:
Elements.attr() iterates on its element to look for the first one with the given attrbute.
If I try to get the attribute abs:href, the test element.hasAttr("abs:herf") fails, and the returned value is an empty string.
```
You are a professional Java test case writer, please create a test case named `handlesAbsPrefixOnHasAttr` for the issue `Jsoup-97`, utilizing the provided issue report information and the following function signature.
```java
@Test public void handlesAbsPrefixOnHasAttr() {
```
| 48 | [
"org.jsoup.nodes.Node"
] | 76f47fdb89bf1d44aa10d10a94bb5e278d374722bb40b1cf73dfe7652499e45a | @Test public void handlesAbsPrefixOnHasAttr() | // You are a professional Java test case writer, please create a test case named `handlesAbsPrefixOnHasAttr` for the issue `Jsoup-97`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Jsoup-97
//
// ## Issue-Title:
// abs: attribute prefix does not work on Elements.attr()
//
// ## Issue-Description:
// Elements.attr() iterates on its element to look for the first one with the given attrbute.
//
//
// If I try to get the attribute abs:href, the test element.hasAttr("abs:herf") fails, and the returned value is an empty string.
//
//
//
//
| Jsoup | package org.jsoup.nodes;
import org.jsoup.Jsoup;
import org.jsoup.TextUtil;
import org.jsoup.parser.Tag;
import org.junit.Test;
import static org.junit.Assert.*;
/**
Tests Nodes
@author Jonathan Hedley, [email protected] */
public class NodeTest {
@Test public void handlesBaseUri() {
Tag tag = Tag.valueOf("a");
Attributes attribs = new Attributes();
attribs.put("relHref", "/foo");
attribs.put("absHref", "http://bar/qux");
Element noBase = new Element(tag, "", attribs);
assertEquals("", noBase.absUrl("relHref")); // with no base, should NOT fallback to href attrib, whatever it is
assertEquals("http://bar/qux", noBase.absUrl("absHref")); // no base but valid attrib, return attrib
Element withBase = new Element(tag, "http://foo/", attribs);
assertEquals("http://foo/foo", withBase.absUrl("relHref")); // construct abs from base + rel
assertEquals("http://bar/qux", withBase.absUrl("absHref")); // href is abs, so returns that
assertEquals("", withBase.absUrl("noval"));
Element dodgyBase = new Element(tag, "wtf://no-such-protocol/", attribs);
assertEquals("http://bar/qux", dodgyBase.absUrl("absHref")); // base fails, but href good, so get that
assertEquals("", dodgyBase.absUrl("relHref")); // base fails, only rel href, so return nothing
}
@Test public void handlesAbsPrefix() {
Document doc = Jsoup.parse("<a href=/foo>Hello</a>", "http://jsoup.org/");
Element a = doc.select("a").first();
assertEquals("/foo", a.attr("href"));
assertEquals("http://jsoup.org/foo", a.attr("abs:href"));
assertTrue(a.hasAttr("abs:href"));
}
@Test public void handlesAbsOnImage() {
Document doc = Jsoup.parse("<p><img src=\"/rez/osi_logo.png\" /></p>", "http://jsoup.org/");
Element img = doc.select("img").first();
assertEquals("http://jsoup.org/rez/osi_logo.png", img.attr("abs:src"));
assertEquals(img.absUrl("src"), img.attr("abs:src"));
}
@Test public void handlesAbsPrefixOnHasAttr() {
// 1: no abs url; 2: has abs url
Document doc = Jsoup.parse("<a id=1 href='/foo'>One</a> <a id=2 href='http://jsoup.org/'>Two</a>");
Element one = doc.select("#1").first();
Element two = doc.select("#2").first();
assertFalse(one.hasAttr("abs:href"));
assertTrue(one.hasAttr("href"));
assertEquals("", one.absUrl("href"));
assertTrue(two.hasAttr("abs:href"));
assertTrue(two.hasAttr("href"));
assertEquals("http://jsoup.org/", two.absUrl("href"));
}
@Test public void literalAbsPrefix() {
// if there is a literal attribute "abs:xxx", don't try and make absolute.
Document doc = Jsoup.parse("<a abs:href='odd'>One</a>");
Element el = doc.select("a").first();
assertTrue(el.hasAttr("abs:href"));
assertEquals("odd", el.attr("abs:href"));
}
/*
Test for an issue with Java's abs URL handler.
*/
@Test public void absHandlesRelativeQuery() {
Document doc = Jsoup.parse("<a href='?foo'>One</a> <a href='bar.html?foo'>Two</a>", "http://jsoup.org/path/file?bar");
Element a1 = doc.select("a").first();
assertEquals("http://jsoup.org/path/file?foo", a1.absUrl("href"));
Element a2 = doc.select("a").get(1);
assertEquals("http://jsoup.org/path/bar.html?foo", a2.absUrl("href"));
}
@Test public void testRemove() {
Document doc = Jsoup.parse("<p>One <span>two</span> three</p>");
Element p = doc.select("p").first();
p.childNode(0).remove();
assertEquals("two three", p.text());
assertEquals("<span>two</span> three", TextUtil.stripNewlines(p.html()));
}
@Test public void testReplace() {
Document doc = Jsoup.parse("<p>One <span>two</span> three</p>");
Element p = doc.select("p").first();
Element insert = doc.createElement("em").text("foo");
p.childNode(1).replaceWith(insert);
assertEquals("One <em>foo</em> three", p.html());
}
@Test public void ownerDocument() {
Document doc = Jsoup.parse("<p>Hello");
Element p = doc.select("p").first();
assertTrue(p.ownerDocument() == doc);
assertTrue(doc.ownerDocument() == doc);
assertNull(doc.parent());
}
@Test public void before() {
Document doc = Jsoup.parse("<p>One <b>two</b> three</p>");
Element newNode = new Element(Tag.valueOf("em"), "");
newNode.appendText("four");
doc.select("b").first().before(newNode);
assertEquals("<p>One <em>four</em><b>two</b> three</p>", doc.body().html());
doc.select("b").first().before("<i>five</i>");
assertEquals("<p>One <em>four</em><i>five</i><b>two</b> three</p>", doc.body().html());
}
@Test public void after() {
Document doc = Jsoup.parse("<p>One <b>two</b> three</p>");
Element newNode = new Element(Tag.valueOf("em"), "");
newNode.appendText("four");
doc.select("b").first().after(newNode);
assertEquals("<p>One <b>two</b><em>four</em> three</p>", doc.body().html());
doc.select("b").first().after("<i>five</i>");
assertEquals("<p>One <b>two</b><i>five</i><em>four</em> three</p>", doc.body().html());
}
} |
||
public void testSingletonGetter1() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.ADVANCED_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
options.setCodingConvention(new ClosureCodingConvention());
test(options,
"/** @const */\n" +
"var goog = goog || {};\n" +
"goog.addSingletonGetter = function(ctor) {\n" +
" ctor.getInstance = function() {\n" +
" return ctor.instance_ || (ctor.instance_ = new ctor());\n" +
" };\n" +
"};" +
"function Foo() {}\n" +
"goog.addSingletonGetter(Foo);" +
"Foo.prototype.bar = 1;" +
"function Bar() {}\n" +
"goog.addSingletonGetter(Bar);" +
"Bar.prototype.bar = 1;",
"");
} | com.google.javascript.jscomp.IntegrationTest::testSingletonGetter1 | test/com/google/javascript/jscomp/IntegrationTest.java | 1,956 | test/com/google/javascript/jscomp/IntegrationTest.java | testSingletonGetter1 | /*
* Copyright 2009 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import com.google.common.base.Joiner;
import com.google.common.collect.ArrayListMultimap;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.Sets;
import com.google.javascript.jscomp.CompilerOptions.LanguageMode;
import com.google.javascript.rhino.Node;
import com.google.javascript.rhino.Token;
import junit.framework.TestCase;
/**
* Tests for {@link PassFactory}.
*
* @author [email protected] (Nick Santos)
*/
public class IntegrationTest extends TestCase {
/** Externs for the test */
private final JSSourceFile[] DEFAULT_EXTERNS = new JSSourceFile[] {
JSSourceFile.fromCode("externs",
"var arguments;\n"
+ "/** @constructor */ function Window() {}\n"
+ "/** @type {string} */ Window.prototype.name;\n"
+ "/** @type {string} */ Window.prototype.offsetWidth;\n"
+ "/** @type {Window} */ var window;\n"
+ "/** @nosideeffects */ function noSideEffects() {}\n"
+ "/** @constructor\n * @nosideeffects */ function Widget() {}\n"
+ "/** @modifies {this} */ Widget.prototype.go = function() {};\n"
+ "/** @return {string} */ var widgetToken = function() {};\n")
};
private JSSourceFile[] externs = DEFAULT_EXTERNS;
private static final String CLOSURE_BOILERPLATE =
"/** @define {boolean} */ var COMPILED = false; var goog = {};" +
"goog.exportSymbol = function() {};";
private static final String CLOSURE_COMPILED =
"var COMPILED = true; var goog$exportSymbol = function() {};";
// The most recently used compiler.
private Compiler lastCompiler;
@Override
public void setUp() {
externs = DEFAULT_EXTERNS;
lastCompiler = null;
}
public void testBug1949424() {
CompilerOptions options = createCompilerOptions();
options.collapseProperties = true;
options.closurePass = true;
test(options, CLOSURE_BOILERPLATE + "goog.provide('FOO'); FOO.bar = 3;",
CLOSURE_COMPILED + "var FOO$bar = 3;");
}
public void testBug1949424_v2() {
CompilerOptions options = createCompilerOptions();
options.collapseProperties = true;
options.closurePass = true;
test(options, CLOSURE_BOILERPLATE + "goog.provide('FOO.BAR'); FOO.BAR = 3;",
CLOSURE_COMPILED + "var FOO$BAR = 3;");
}
public void testBug1956277() {
CompilerOptions options = createCompilerOptions();
options.collapseProperties = true;
options.inlineVariables = true;
test(options, "var CONST = {}; CONST.bar = null;" +
"function f(url) { CONST.bar = url; }",
"var CONST$bar = null; function f(url) { CONST$bar = url; }");
}
public void testBug1962380() {
CompilerOptions options = createCompilerOptions();
options.collapseProperties = true;
options.inlineVariables = true;
options.generateExports = true;
test(options,
CLOSURE_BOILERPLATE + "/** @export */ goog.CONSTANT = 1;" +
"var x = goog.CONSTANT;",
"(function() {})('goog.CONSTANT', 1);" +
"var x = 1;");
}
public void testBug2410122() {
CompilerOptions options = createCompilerOptions();
options.generateExports = true;
options.closurePass = true;
test(options,
"var goog = {};" +
"function F() {}" +
"/** @export */ function G() { goog.base(this); } " +
"goog.inherits(G, F);",
"var goog = {};" +
"function F() {}" +
"function G() { F.call(this); } " +
"goog.inherits(G, F); goog.exportSymbol('G', G);");
}
public void testIssue90() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
options.inlineVariables = true;
options.removeDeadCode = true;
test(options,
"var x; x && alert(1);",
"");
}
public void testClosurePassOff() {
CompilerOptions options = createCompilerOptions();
options.closurePass = false;
testSame(
options,
"var goog = {}; goog.require = function(x) {}; goog.require('foo');");
testSame(
options,
"var goog = {}; goog.getCssName = function(x) {};" +
"goog.getCssName('foo');");
}
public void testClosurePassOn() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
test(
options,
"var goog = {}; goog.require = function(x) {}; goog.require('foo');",
ProcessClosurePrimitives.MISSING_PROVIDE_ERROR);
test(
options,
"/** @define {boolean} */ var COMPILED = false;" +
"var goog = {}; goog.getCssName = function(x) {};" +
"goog.getCssName('foo');",
"var COMPILED = true;" +
"var goog = {}; goog.getCssName = function(x) {};" +
"'foo';");
}
public void testCssNameCheck() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.checkMissingGetCssNameLevel = CheckLevel.ERROR;
options.checkMissingGetCssNameBlacklist = "foo";
test(options, "var x = 'foo';",
CheckMissingGetCssName.MISSING_GETCSSNAME);
}
public void testBug2592659() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.checkTypes = true;
options.checkMissingGetCssNameLevel = CheckLevel.WARNING;
options.checkMissingGetCssNameBlacklist = "foo";
test(options,
"var goog = {};\n" +
"/**\n" +
" * @param {string} className\n" +
" * @param {string=} opt_modifier\n" +
" * @return {string}\n" +
"*/\n" +
"goog.getCssName = function(className, opt_modifier) {}\n" +
"var x = goog.getCssName(123, 'a');",
TypeValidator.TYPE_MISMATCH_WARNING);
}
public void testTypedefBeforeOwner1() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
test(options,
"goog.provide('foo.Bar.Type');\n" +
"goog.provide('foo.Bar');\n" +
"/** @typedef {number} */ foo.Bar.Type;\n" +
"foo.Bar = function() {};",
"var foo = {}; foo.Bar.Type; foo.Bar = function() {};");
}
public void testTypedefBeforeOwner2() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.collapseProperties = true;
test(options,
"goog.provide('foo.Bar.Type');\n" +
"goog.provide('foo.Bar');\n" +
"/** @typedef {number} */ foo.Bar.Type;\n" +
"foo.Bar = function() {};",
"var foo$Bar$Type; var foo$Bar = function() {};");
}
public void testExportedNames() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.variableRenaming = VariableRenamingPolicy.ALL;
test(options,
"/** @define {boolean} */ var COMPILED = false;" +
"var goog = {}; goog.exportSymbol('b', goog);",
"var a = true; var c = {}; c.exportSymbol('b', c);");
test(options,
"/** @define {boolean} */ var COMPILED = false;" +
"var goog = {}; goog.exportSymbol('a', goog);",
"var b = true; var c = {}; c.exportSymbol('a', c);");
}
public void testCheckGlobalThisOn() {
CompilerOptions options = createCompilerOptions();
options.checkSuspiciousCode = true;
options.checkGlobalThisLevel = CheckLevel.ERROR;
test(options, "function f() { this.y = 3; }", CheckGlobalThis.GLOBAL_THIS);
}
public void testSusiciousCodeOff() {
CompilerOptions options = createCompilerOptions();
options.checkSuspiciousCode = false;
options.checkGlobalThisLevel = CheckLevel.ERROR;
test(options, "function f() { this.y = 3; }", CheckGlobalThis.GLOBAL_THIS);
}
public void testCheckGlobalThisOff() {
CompilerOptions options = createCompilerOptions();
options.checkSuspiciousCode = true;
options.checkGlobalThisLevel = CheckLevel.OFF;
testSame(options, "function f() { this.y = 3; }");
}
public void testCheckRequiresAndCheckProvidesOff() {
testSame(createCompilerOptions(), new String[] {
"/** @constructor */ function Foo() {}",
"new Foo();"
});
}
public void testCheckRequiresOn() {
CompilerOptions options = createCompilerOptions();
options.checkRequires = CheckLevel.ERROR;
test(options, new String[] {
"/** @constructor */ function Foo() {}",
"new Foo();"
}, CheckRequiresForConstructors.MISSING_REQUIRE_WARNING);
}
public void testCheckProvidesOn() {
CompilerOptions options = createCompilerOptions();
options.checkProvides = CheckLevel.ERROR;
test(options, new String[] {
"/** @constructor */ function Foo() {}",
"new Foo();"
}, CheckProvides.MISSING_PROVIDE_WARNING);
}
public void testGenerateExportsOff() {
testSame(createCompilerOptions(), "/** @export */ function f() {}");
}
public void testGenerateExportsOn() {
CompilerOptions options = createCompilerOptions();
options.generateExports = true;
test(options, "/** @export */ function f() {}",
"/** @export */ function f() {} goog.exportSymbol('f', f);");
}
public void testExportTestFunctionsOff() {
testSame(createCompilerOptions(), "function testFoo() {}");
}
public void testExportTestFunctionsOn() {
CompilerOptions options = createCompilerOptions();
options.exportTestFunctions = true;
test(options, "function testFoo() {}",
"/** @export */ function testFoo() {}" +
"goog.exportSymbol('testFoo', testFoo);");
}
public void testCheckSymbolsOff() {
CompilerOptions options = createCompilerOptions();
testSame(options, "x = 3;");
}
public void testCheckSymbolsOn() {
CompilerOptions options = createCompilerOptions();
options.checkSymbols = true;
test(options, "x = 3;", VarCheck.UNDEFINED_VAR_ERROR);
}
public void testCheckReferencesOff() {
CompilerOptions options = createCompilerOptions();
testSame(options, "x = 3; var x = 5;");
}
public void testCheckReferencesOn() {
CompilerOptions options = createCompilerOptions();
options.aggressiveVarCheck = CheckLevel.ERROR;
test(options, "x = 3; var x = 5;",
VariableReferenceCheck.UNDECLARED_REFERENCE);
}
public void testInferTypes() {
CompilerOptions options = createCompilerOptions();
options.inferTypes = true;
options.checkTypes = false;
options.closurePass = true;
test(options,
CLOSURE_BOILERPLATE +
"goog.provide('Foo'); /** @enum */ Foo = {a: 3};",
TypeCheck.ENUM_NOT_CONSTANT);
assertTrue(lastCompiler.getErrorManager().getTypedPercent() == 0);
// This does not generate a warning.
test(options, "/** @type {number} */ var n = window.name;",
"var n = window.name;");
assertTrue(lastCompiler.getErrorManager().getTypedPercent() == 0);
}
public void testTypeCheckAndInference() {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
test(options, "/** @type {number} */ var n = window.name;",
TypeValidator.TYPE_MISMATCH_WARNING);
assertTrue(lastCompiler.getErrorManager().getTypedPercent() > 0);
}
public void testTypeNameParser() {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
test(options, "/** @type {n} */ var n = window.name;",
RhinoErrorReporter.TYPE_PARSE_ERROR);
}
// This tests that the TypedScopeCreator is memoized so that it only creates a
// Scope object once for each scope. If, when type inference requests a scope,
// it creates a new one, then multiple JSType objects end up getting created
// for the same local type, and ambiguate will rename the last statement to
// o.a(o.a, o.a), which is bad.
public void testMemoizedTypedScopeCreator() {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
options.ambiguateProperties = true;
options.propertyRenaming = PropertyRenamingPolicy.ALL_UNQUOTED;
test(options, "function someTest() {\n"
+ " /** @constructor */\n"
+ " function Foo() { this.instProp = 3; }\n"
+ " Foo.prototype.protoProp = function(a, b) {};\n"
+ " /** @constructor\n @extends Foo */\n"
+ " function Bar() {}\n"
+ " goog.inherits(Bar, Foo);\n"
+ " var o = new Bar();\n"
+ " o.protoProp(o.protoProp, o.instProp);\n"
+ "}",
"function someTest() {\n"
+ " function Foo() { this.b = 3; }\n"
+ " Foo.prototype.a = function(a, b) {};\n"
+ " function Bar() {}\n"
+ " goog.c(Bar, Foo);\n"
+ " var o = new Bar();\n"
+ " o.a(o.a, o.b);\n"
+ "}");
}
public void testCheckTypes() {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
test(options, "var x = x || {}; x.f = function() {}; x.f(3);",
TypeCheck.WRONG_ARGUMENT_COUNT);
}
public void testReplaceCssNames() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.gatherCssNames = true;
test(options, "/** @define {boolean} */\n"
+ "var COMPILED = false;\n"
+ "goog.setCssNameMapping({'foo':'bar'});\n"
+ "function getCss() {\n"
+ " return goog.getCssName('foo');\n"
+ "}",
"var COMPILED = true;\n"
+ "function getCss() {\n"
+ " return \"bar\";"
+ "}");
assertEquals(
ImmutableMap.of("foo", new Integer(1)),
lastCompiler.getPassConfig().getIntermediateState().cssNames);
}
public void testRemoveClosureAsserts() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
testSame(options,
"var goog = {};"
+ "goog.asserts.assert(goog);");
options.removeClosureAsserts = true;
test(options,
"var goog = {};"
+ "goog.asserts.assert(goog);",
"var goog = {};");
}
public void testDeprecation() {
String code = "/** @deprecated */ function f() { } function g() { f(); }";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.setWarningLevel(DiagnosticGroups.DEPRECATED, CheckLevel.ERROR);
testSame(options, code);
options.checkTypes = true;
test(options, code, CheckAccessControls.DEPRECATED_NAME);
}
public void testVisibility() {
String[] code = {
"/** @private */ function f() { }",
"function g() { f(); }"
};
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.setWarningLevel(DiagnosticGroups.VISIBILITY, CheckLevel.ERROR);
testSame(options, code);
options.checkTypes = true;
test(options, code, CheckAccessControls.BAD_PRIVATE_GLOBAL_ACCESS);
}
public void testUnreachableCode() {
String code = "function f() { return \n 3; }";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.checkUnreachableCode = CheckLevel.ERROR;
test(options, code, CheckUnreachableCode.UNREACHABLE_CODE);
}
public void testMissingReturn() {
String code =
"/** @return {number} */ function f() { if (f) { return 3; } }";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.checkMissingReturn = CheckLevel.ERROR;
testSame(options, code);
options.checkTypes = true;
test(options, code, CheckMissingReturn.MISSING_RETURN_STATEMENT);
}
public void testIdGenerators() {
String code = "function f() {} f('id');";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.idGenerators = Sets.newHashSet("f");
test(options, code, "function f() {} 'a';");
}
public void testOptimizeArgumentsArray() {
String code = "function f() { return arguments[0]; }";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.optimizeArgumentsArray = true;
String argName = "JSCompiler_OptimizeArgumentsArray_p0";
test(options, code,
"function f(" + argName + ") { return " + argName + "; }");
}
public void testOptimizeParameters() {
String code = "function f(a) { return a; } f(true);";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.optimizeParameters = true;
test(options, code, "function f() { var a = true; return a;} f();");
}
public void testOptimizeReturns() {
String code = "function f(a) { return a; } f(true);";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.optimizeReturns = true;
test(options, code, "function f(a) {return;} f(true);");
}
public void testRemoveAbstractMethods() {
String code = CLOSURE_BOILERPLATE +
"var x = {}; x.foo = goog.abstractMethod; x.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.closurePass = true;
options.collapseProperties = true;
test(options, code, CLOSURE_COMPILED + " var x$bar = 3;");
}
public void testCollapseProperties1() {
String code =
"var x = {}; x.FOO = 5; x.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.collapseProperties = true;
test(options, code, "var x$FOO = 5; var x$bar = 3;");
}
public void testCollapseProperties2() {
String code =
"var x = {}; x.FOO = 5; x.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.collapseProperties = true;
options.collapseObjectLiterals = true;
test(options, code, "var x$FOO = 5; var x$bar = 3;");
}
public void testCollapseObjectLiteral1() {
// Verify collapseObjectLiterals does nothing in global scope
String code = "var x = {}; x.FOO = 5; x.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.collapseObjectLiterals = true;
testSame(options, code);
}
public void testCollapseObjectLiteral2() {
String code =
"function f() {var x = {}; x.FOO = 5; x.bar = 3;}";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.collapseObjectLiterals = true;
test(options, code,
"function f(){" +
"var JSCompiler_object_inline_FOO_0;" +
"var JSCompiler_object_inline_bar_1;" +
"JSCompiler_object_inline_FOO_0=5;" +
"JSCompiler_object_inline_bar_1=3}");
}
public void testTightenTypesWithoutTypeCheck() {
CompilerOptions options = createCompilerOptions();
options.tightenTypes = true;
test(options, "", DefaultPassConfig.TIGHTEN_TYPES_WITHOUT_TYPE_CHECK);
}
public void testDisambiguateProperties() {
String code =
"/** @constructor */ function Foo(){} Foo.prototype.bar = 3;" +
"/** @constructor */ function Baz(){} Baz.prototype.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.disambiguateProperties = true;
options.checkTypes = true;
test(options, code,
"function Foo(){} Foo.prototype.Foo_prototype$bar = 3;" +
"function Baz(){} Baz.prototype.Baz_prototype$bar = 3;");
}
public void testMarkPureCalls() {
String testCode = "function foo() {} foo();";
CompilerOptions options = createCompilerOptions();
options.removeDeadCode = true;
testSame(options, testCode);
options.computeFunctionSideEffects = true;
test(options, testCode, "function foo() {}");
}
public void testMarkNoSideEffects() {
String testCode = "noSideEffects();";
CompilerOptions options = createCompilerOptions();
options.removeDeadCode = true;
testSame(options, testCode);
options.markNoSideEffectCalls = true;
test(options, testCode, "");
}
public void testChainedCalls() {
CompilerOptions options = createCompilerOptions();
options.chainCalls = true;
test(
options,
"/** @constructor */ function Foo() {} " +
"Foo.prototype.bar = function() { return this; }; " +
"var f = new Foo();" +
"f.bar(); " +
"f.bar(); ",
"function Foo() {} " +
"Foo.prototype.bar = function() { return this; }; " +
"var f = new Foo();" +
"f.bar().bar();");
}
public void testExtraAnnotationNames() {
CompilerOptions options = createCompilerOptions();
options.setExtraAnnotationNames(Sets.newHashSet("TagA", "TagB"));
test(
options,
"/** @TagA */ var f = new Foo(); /** @TagB */ f.bar();",
"var f = new Foo(); f.bar();");
}
public void testDevirtualizePrototypeMethods() {
CompilerOptions options = createCompilerOptions();
options.devirtualizePrototypeMethods = true;
test(
options,
"/** @constructor */ var Foo = function() {}; " +
"Foo.prototype.bar = function() {};" +
"(new Foo()).bar();",
"var Foo = function() {};" +
"var JSCompiler_StaticMethods_bar = " +
" function(JSCompiler_StaticMethods_bar$self) {};" +
"JSCompiler_StaticMethods_bar(new Foo());");
}
public void testCheckConsts() {
CompilerOptions options = createCompilerOptions();
options.inlineConstantVars = true;
test(options, "var FOO = true; FOO = false",
ConstCheck.CONST_REASSIGNED_VALUE_ERROR);
}
public void testAllChecksOn() {
CompilerOptions options = createCompilerOptions();
options.checkSuspiciousCode = true;
options.checkControlStructures = true;
options.checkRequires = CheckLevel.ERROR;
options.checkProvides = CheckLevel.ERROR;
options.generateExports = true;
options.exportTestFunctions = true;
options.closurePass = true;
options.checkMissingGetCssNameLevel = CheckLevel.ERROR;
options.checkMissingGetCssNameBlacklist = "goog";
options.syntheticBlockStartMarker = "synStart";
options.syntheticBlockEndMarker = "synEnd";
options.checkSymbols = true;
options.aggressiveVarCheck = CheckLevel.ERROR;
options.processObjectPropertyString = true;
options.collapseProperties = true;
test(options, CLOSURE_BOILERPLATE, CLOSURE_COMPILED);
}
public void testTypeCheckingWithSyntheticBlocks() {
CompilerOptions options = createCompilerOptions();
options.syntheticBlockStartMarker = "synStart";
options.syntheticBlockEndMarker = "synEnd";
options.checkTypes = true;
// We used to have a bug where the CFG drew an
// edge straight from synStart to f(progress).
// If that happens, then progress will get type {number|undefined}.
testSame(
options,
"/** @param {number} x */ function f(x) {}" +
"function g() {" +
" synStart('foo');" +
" var progress = 1;" +
" f(progress);" +
" synEnd('foo');" +
"}");
}
public void testCompilerDoesNotBlowUpIfUndefinedSymbols() {
CompilerOptions options = createCompilerOptions();
options.checkSymbols = true;
// Disable the undefined variable check.
options.setWarningLevel(
DiagnosticGroup.forType(VarCheck.UNDEFINED_VAR_ERROR),
CheckLevel.OFF);
// The compiler used to throw an IllegalStateException on this.
testSame(options, "var x = {foo: y};");
}
// Make sure that if we change variables which are constant to have
// $$constant appended to their names, we remove that tag before
// we finish.
public void testConstantTagsMustAlwaysBeRemoved() {
CompilerOptions options = createCompilerOptions();
options.variableRenaming = VariableRenamingPolicy.LOCAL;
String originalText = "var G_GEO_UNKNOWN_ADDRESS=1;\n" +
"function foo() {" +
" var localVar = 2;\n" +
" if (G_GEO_UNKNOWN_ADDRESS == localVar) {\n" +
" alert(\"A\"); }}";
String expectedText = "var G_GEO_UNKNOWN_ADDRESS=1;" +
"function foo(){var a=2;if(G_GEO_UNKNOWN_ADDRESS==a){alert(\"A\")}}";
test(options, originalText, expectedText);
}
public void testClosurePassPreservesJsDoc() {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
options.closurePass = true;
test(options,
CLOSURE_BOILERPLATE +
"goog.provide('Foo'); /** @constructor */ Foo = function() {};" +
"var x = new Foo();",
"var COMPILED=true;var goog={};goog.exportSymbol=function(){};" +
"var Foo=function(){};var x=new Foo");
test(options,
CLOSURE_BOILERPLATE +
"goog.provide('Foo'); /** @enum */ Foo = {a: 3};",
TypeCheck.ENUM_NOT_CONSTANT);
}
public void testProvidedNamespaceIsConst() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.inlineConstantVars = true;
options.collapseProperties = true;
test(options,
"var goog = {}; goog.provide('foo'); " +
"function f() { foo = {};}",
"var foo = {}; function f() { foo = {}; }",
ConstCheck.CONST_REASSIGNED_VALUE_ERROR);
}
public void testProvidedNamespaceIsConst2() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.inlineConstantVars = true;
options.collapseProperties = true;
test(options,
"var goog = {}; goog.provide('foo.bar'); " +
"function f() { foo.bar = {};}",
"var foo$bar = {};" +
"function f() { foo$bar = {}; }",
ConstCheck.CONST_REASSIGNED_VALUE_ERROR);
}
public void testProvidedNamespaceIsConst3() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.inlineConstantVars = true;
options.collapseProperties = true;
test(options,
"var goog = {}; " +
"goog.provide('foo.bar'); goog.provide('foo.bar.baz'); " +
"/** @constructor */ foo.bar = function() {};" +
"/** @constructor */ foo.bar.baz = function() {};",
"var foo$bar = function(){};" +
"var foo$bar$baz = function(){};");
}
public void testProvidedNamespaceIsConst4() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.inlineConstantVars = true;
options.collapseProperties = true;
test(options,
"var goog = {}; goog.provide('foo.Bar'); " +
"var foo = {}; foo.Bar = {};",
"var foo = {}; var foo = {}; foo.Bar = {};");
}
public void testProvidedNamespaceIsConst5() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.inlineConstantVars = true;
options.collapseProperties = true;
test(options,
"var goog = {}; goog.provide('foo.Bar'); " +
"foo = {}; foo.Bar = {};",
"var foo = {}; foo = {}; foo.Bar = {};");
}
public void testProcessDefinesAlwaysOn() {
test(createCompilerOptions(),
"/** @define {boolean} */ var HI = true; HI = false;",
"var HI = false;false;");
}
public void testProcessDefinesAdditionalReplacements() {
CompilerOptions options = createCompilerOptions();
options.setDefineToBooleanLiteral("HI", false);
test(options,
"/** @define {boolean} */ var HI = true;",
"var HI = false;");
}
public void testReplaceMessages() {
CompilerOptions options = createCompilerOptions();
String prefix = "var goog = {}; goog.getMsg = function() {};";
testSame(options, prefix + "var MSG_HI = goog.getMsg('hi');");
options.messageBundle = new EmptyMessageBundle();
test(options,
prefix + "/** @desc xyz */ var MSG_HI = goog.getMsg('hi');",
prefix + "var MSG_HI = 'hi';");
}
public void testCheckGlobalNames() {
CompilerOptions options = createCompilerOptions();
options.checkGlobalNamesLevel = CheckLevel.ERROR;
test(options, "var x = {}; var y = x.z;",
CheckGlobalNames.UNDEFINED_NAME_WARNING);
}
public void testInlineGetters() {
CompilerOptions options = createCompilerOptions();
String code =
"function Foo() {} Foo.prototype.bar = function() { return 3; };" +
"var x = new Foo(); x.bar();";
testSame(options, code);
options.inlineGetters = true;
test(options, code,
"function Foo() {} Foo.prototype.bar = function() { return 3 };" +
"var x = new Foo(); 3;");
}
public void testInlineGettersWithAmbiguate() {
CompilerOptions options = createCompilerOptions();
String code =
"/** @constructor */" +
"function Foo() {}" +
"/** @type {number} */ Foo.prototype.field;" +
"Foo.prototype.getField = function() { return this.field; };" +
"/** @constructor */" +
"function Bar() {}" +
"/** @type {string} */ Bar.prototype.field;" +
"Bar.prototype.getField = function() { return this.field; };" +
"new Foo().getField();" +
"new Bar().getField();";
testSame(options, code);
options.inlineGetters = true;
test(options, code,
"function Foo() {}" +
"Foo.prototype.field;" +
"Foo.prototype.getField = function() { return this.field; };" +
"function Bar() {}" +
"Bar.prototype.field;" +
"Bar.prototype.getField = function() { return this.field; };" +
"new Foo().field;" +
"new Bar().field;");
options.checkTypes = true;
options.ambiguateProperties = true;
// Propagating the wrong type information may cause ambiguate properties
// to generate bad code.
testSame(options, code);
}
public void testInlineVariables() {
CompilerOptions options = createCompilerOptions();
String code = "function foo() {} var x = 3; foo(x);";
testSame(options, code);
options.inlineVariables = true;
test(options, code, "(function foo() {})(3);");
options.propertyRenaming = PropertyRenamingPolicy.HEURISTIC;
test(options, code, DefaultPassConfig.CANNOT_USE_PROTOTYPE_AND_VAR);
}
public void testInlineConstants() {
CompilerOptions options = createCompilerOptions();
String code = "function foo() {} var x = 3; foo(x); var YYY = 4; foo(YYY);";
testSame(options, code);
options.inlineConstantVars = true;
test(options, code, "function foo() {} var x = 3; foo(x); foo(4);");
}
public void testMinimizeExits() {
CompilerOptions options = createCompilerOptions();
String code =
"function f() {" +
" if (window.foo) return; window.h(); " +
"}";
testSame(options, code);
options.foldConstants = true;
test(
options, code,
"function f() {" +
" window.foo || window.h(); " +
"}");
}
public void testFoldConstants() {
CompilerOptions options = createCompilerOptions();
String code = "if (true) { window.foo(); }";
testSame(options, code);
options.foldConstants = true;
test(options, code, "window.foo();");
}
public void testRemoveUnreachableCode() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { return; f(); }";
testSame(options, code);
options.removeDeadCode = true;
test(options, code, "function f() {}");
}
public void testRemoveUnusedPrototypeProperties1() {
CompilerOptions options = createCompilerOptions();
String code = "function Foo() {} " +
"Foo.prototype.bar = function() { return new Foo(); };";
testSame(options, code);
options.removeUnusedPrototypeProperties = true;
test(options, code, "function Foo() {}");
}
public void testRemoveUnusedPrototypeProperties2() {
CompilerOptions options = createCompilerOptions();
String code = "function Foo() {} " +
"Foo.prototype.bar = function() { return new Foo(); };" +
"function f(x) { x.bar(); }";
testSame(options, code);
options.removeUnusedPrototypeProperties = true;
testSame(options, code);
options.removeUnusedVars = true;
test(options, code, "");
}
public void testSmartNamePass() {
CompilerOptions options = createCompilerOptions();
String code = "function Foo() { this.bar(); } " +
"Foo.prototype.bar = function() { return Foo(); };";
testSame(options, code);
options.smartNameRemoval = true;
test(options, code, "");
}
public void testDeadAssignmentsElimination() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { var x = 3; 4; x = 5; return x; } f(); ";
testSame(options, code);
options.deadAssignmentElimination = true;
testSame(options, code);
options.removeUnusedVars = true;
test(options, code, "function f() { var x = 3; 4; x = 5; return x; } f();");
}
public void testInlineFunctions() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { return 3; } f(); ";
testSame(options, code);
options.inlineFunctions = true;
test(options, code, "3;");
}
public void testRemoveUnusedVars1() {
CompilerOptions options = createCompilerOptions();
String code = "function f(x) {} f();";
testSame(options, code);
options.removeUnusedVars = true;
test(options, code, "function f() {} f();");
}
public void testRemoveUnusedVars2() {
CompilerOptions options = createCompilerOptions();
String code = "(function f(x) {})();var g = function() {}; g();";
testSame(options, code);
options.removeUnusedVars = true;
test(options, code, "(function() {})();var g = function() {}; g();");
options.anonymousFunctionNaming = AnonymousFunctionNamingPolicy.UNMAPPED;
test(options, code, "(function f() {})();var g = function $g$() {}; g();");
}
public void testCrossModuleCodeMotion() {
CompilerOptions options = createCompilerOptions();
String[] code = new String[] {
"var x = 1;",
"x;",
};
testSame(options, code);
options.crossModuleCodeMotion = true;
test(options, code, new String[] {
"",
"var x = 1; x;",
});
}
public void testCrossModuleMethodMotion() {
CompilerOptions options = createCompilerOptions();
String[] code = new String[] {
"var Foo = function() {}; Foo.prototype.bar = function() {};" +
"var x = new Foo();",
"x.bar();",
};
testSame(options, code);
options.crossModuleMethodMotion = true;
test(options, code, new String[] {
CrossModuleMethodMotion.STUB_DECLARATIONS +
"var Foo = function() {};" +
"Foo.prototype.bar=JSCompiler_stubMethod(0); var x=new Foo;",
"Foo.prototype.bar=JSCompiler_unstubMethod(0,function(){}); x.bar()",
});
}
public void testFlowSensitiveInlineVariables1() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { var x = 3; x = 5; return x; }";
testSame(options, code);
options.flowSensitiveInlineVariables = true;
test(options, code, "function f() { var x = 3; return 5; }");
String unusedVar = "function f() { var x; x = 5; return x; } f()";
test(options, unusedVar, "function f() { var x; return 5; } f()");
options.removeUnusedVars = true;
test(options, unusedVar, "function f() { return 5; } f()");
}
public void testFlowSensitiveInlineVariables2() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.SIMPLE_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
test(options,
"function f () {\n" +
" var ab = 0;\n" +
" ab += '-';\n" +
" alert(ab);\n" +
"}",
"function f () {\n" +
" alert('0-');\n" +
"}");
}
public void testCollapseAnonymousFunctions() {
CompilerOptions options = createCompilerOptions();
String code = "var f = function() {};";
testSame(options, code);
options.collapseAnonymousFunctions = true;
test(options, code, "function f() {}");
}
public void testMoveFunctionDeclarations() {
CompilerOptions options = createCompilerOptions();
String code = "var x = f(); function f() { return 3; }";
testSame(options, code);
options.moveFunctionDeclarations = true;
test(options, code, "function f() { return 3; } var x = f();");
}
public void testNameAnonymousFunctions() {
CompilerOptions options = createCompilerOptions();
String code = "var f = function() {};";
testSame(options, code);
options.anonymousFunctionNaming = AnonymousFunctionNamingPolicy.MAPPED;
test(options, code, "var f = function $() {}");
assertNotNull(lastCompiler.getResult().namedAnonFunctionMap);
options.anonymousFunctionNaming = AnonymousFunctionNamingPolicy.UNMAPPED;
test(options, code, "var f = function $f$() {}");
assertNull(lastCompiler.getResult().namedAnonFunctionMap);
}
public void testExtractPrototypeMemberDeclarations() {
CompilerOptions options = createCompilerOptions();
String code = "var f = function() {};";
String expected = "var a; var b = function() {}; a = b.prototype;";
for (int i = 0; i < 10; i++) {
code += "f.prototype.a = " + i + ";";
expected += "a.a = " + i + ";";
}
testSame(options, code);
options.extractPrototypeMemberDeclarations = true;
options.variableRenaming = VariableRenamingPolicy.ALL;
test(options, code, expected);
options.propertyRenaming = PropertyRenamingPolicy.HEURISTIC;
options.variableRenaming = VariableRenamingPolicy.OFF;
testSame(options, code);
}
public void testDevirtualizationAndExtractPrototypeMemberDeclarations() {
CompilerOptions options = createCompilerOptions();
options.devirtualizePrototypeMethods = true;
options.collapseAnonymousFunctions = true;
options.extractPrototypeMemberDeclarations = true;
options.variableRenaming = VariableRenamingPolicy.ALL;
String code = "var f = function() {};";
String expected = "var a; function b() {} a = b.prototype;";
for (int i = 0; i < 10; i++) {
code += "f.prototype.argz = function() {arguments};";
code += "f.prototype.devir" + i + " = function() {};";
char letter = (char) ('d' + i);
expected += "a.argz = function() {arguments};";
expected += "function " + letter + "(c){}";
}
code += "var F = new f(); F.argz();";
expected += "var n = new b(); n.argz();";
for (int i = 0; i < 10; i++) {
code += "F.devir" + i + "();";
char letter = (char) ('d' + i);
expected += letter + "(n);";
}
test(options, code, expected);
}
public void testCoalesceVariableNames() {
CompilerOptions options = createCompilerOptions();
String code = "function f() {var x = 3; var y = x; var z = y; return z;}";
testSame(options, code);
options.coalesceVariableNames = true;
test(options, code,
"function f() {var x = 3; x = x; x = x; return x;}");
}
public void testPropertyRenaming() {
CompilerOptions options = createCompilerOptions();
options.propertyAffinity = true;
String code =
"function f() { return this.foo + this['bar'] + this.Baz; }" +
"f.prototype.bar = 3; f.prototype.Baz = 3;";
String heuristic =
"function f() { return this.foo + this['bar'] + this.a; }" +
"f.prototype.bar = 3; f.prototype.a = 3;";
String aggHeuristic =
"function f() { return this.foo + this['b'] + this.a; } " +
"f.prototype.b = 3; f.prototype.a = 3;";
String all =
"function f() { return this.b + this['bar'] + this.a; }" +
"f.prototype.c = 3; f.prototype.a = 3;";
testSame(options, code);
options.propertyRenaming = PropertyRenamingPolicy.HEURISTIC;
test(options, code, heuristic);
options.propertyRenaming = PropertyRenamingPolicy.AGGRESSIVE_HEURISTIC;
test(options, code, aggHeuristic);
options.propertyRenaming = PropertyRenamingPolicy.ALL_UNQUOTED;
test(options, code, all);
}
public void testConvertToDottedProperties() {
CompilerOptions options = createCompilerOptions();
String code =
"function f() { return this['bar']; } f.prototype.bar = 3;";
String expected =
"function f() { return this.bar; } f.prototype.a = 3;";
testSame(options, code);
options.convertToDottedProperties = true;
options.propertyRenaming = PropertyRenamingPolicy.ALL_UNQUOTED;
test(options, code, expected);
}
public void testRewriteFunctionExpressions() {
CompilerOptions options = createCompilerOptions();
String code = "var a = function() {};";
String expected = "function JSCompiler_emptyFn(){return function(){}} " +
"var a = JSCompiler_emptyFn();";
for (int i = 0; i < 10; i++) {
code += "a = function() {};";
expected += "a = JSCompiler_emptyFn();";
}
testSame(options, code);
options.rewriteFunctionExpressions = true;
test(options, code, expected);
}
public void testAliasAllStrings() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { return 'a'; }";
String expected = "var $$S_a = 'a'; function f() { return $$S_a; }";
testSame(options, code);
options.aliasAllStrings = true;
test(options, code, expected);
}
public void testAliasExterns() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { return window + window + window + window; }";
String expected = "var GLOBAL_window = window;" +
"function f() { return GLOBAL_window + GLOBAL_window + " +
" GLOBAL_window + GLOBAL_window; }";
testSame(options, code);
options.aliasExternals = true;
test(options, code, expected);
}
public void testAliasKeywords() {
CompilerOptions options = createCompilerOptions();
String code =
"function f() { return true + true + true + true + true + true; }";
String expected = "var JSCompiler_alias_TRUE = true;" +
"function f() { return JSCompiler_alias_TRUE + " +
" JSCompiler_alias_TRUE + JSCompiler_alias_TRUE + " +
" JSCompiler_alias_TRUE + JSCompiler_alias_TRUE + " +
" JSCompiler_alias_TRUE; }";
testSame(options, code);
options.aliasKeywords = true;
test(options, code, expected);
}
public void testRenameVars1() {
CompilerOptions options = createCompilerOptions();
String code =
"var abc = 3; function f() { var xyz = 5; return abc + xyz; }";
String local = "var abc = 3; function f() { var a = 5; return abc + a; }";
String all = "var a = 3; function c() { var b = 5; return a + b; }";
testSame(options, code);
options.variableRenaming = VariableRenamingPolicy.LOCAL;
test(options, code, local);
options.variableRenaming = VariableRenamingPolicy.ALL;
test(options, code, all);
options.reserveRawExports = true;
}
public void testRenameVars2() {
CompilerOptions options = createCompilerOptions();
options.variableRenaming = VariableRenamingPolicy.ALL;
String code = "var abc = 3; function f() { window['a'] = 5; }";
String noexport = "var a = 3; function b() { window['a'] = 5; }";
String export = "var b = 3; function c() { window['a'] = 5; }";
options.reserveRawExports = false;
test(options, code, noexport);
options.reserveRawExports = true;
test(options, code, export);
}
public void testShadowVaribles() {
CompilerOptions options = createCompilerOptions();
options.variableRenaming = VariableRenamingPolicy.LOCAL;
options.shadowVariables = true;
String code = "var f = function(x) { return function(y) {}}";
String expected = "var f = function(a) { return function(a) {}}";
test(options, code, expected);
}
public void testRenameLabels() {
CompilerOptions options = createCompilerOptions();
String code = "longLabel: while (true) { break longLabel; }";
String expected = "a: while (true) { break a; }";
testSame(options, code);
options.labelRenaming = true;
test(options, code, expected);
}
public void testBadBreakStatementInIdeMode() {
// Ensure that type-checking doesn't crash, even if the CFG is malformed.
// This can happen in IDE mode.
CompilerOptions options = createCompilerOptions();
options.ideMode = true;
options.checkTypes = true;
test(options,
"function f() { try { } catch(e) { break; } }",
RhinoErrorReporter.PARSE_ERROR);
}
public void testIssue63SourceMap() {
CompilerOptions options = createCompilerOptions();
String code = "var a;";
options.skipAllPasses = true;
options.sourceMapOutputPath = "./src.map";
Compiler compiler = compile(options, code);
compiler.toSource();
}
public void testRegExp1() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = "/(a)/.test(\"a\");";
testSame(options, code);
options.computeFunctionSideEffects = true;
String expected = "";
test(options, code, expected);
}
public void testRegExp2() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = "/(a)/.test(\"a\");var a = RegExp.$1";
testSame(options, code);
options.computeFunctionSideEffects = true;
test(options, code, CheckRegExp.REGEXP_REFERENCE);
options.setWarningLevel(DiagnosticGroups.CHECK_REGEXP, CheckLevel.OFF);
testSame(options, code);
}
public void testFoldLocals1() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
// An external object, whose constructor has no side-effects,
// and whose method "go" only modifies the object.
String code = "new Widget().go();";
testSame(options, code);
options.computeFunctionSideEffects = true;
test(options, code, "");
}
public void testFoldLocals2() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
options.checkTypes = true;
// An external function that returns a local object that the
// method "go" that only modifies the object.
String code = "widgetToken().go();";
testSame(options, code);
options.computeFunctionSideEffects = true;
test(options, code, "widgetToken()");
}
public void testFoldLocals3() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
// A function "f" who returns a known local object, and a method that
// modifies only modifies that.
String definition = "function f(){return new Widget()}";
String call = "f().go();";
String code = definition + call;
testSame(options, code);
options.computeFunctionSideEffects = true;
// BROKEN
//test(options, code, definition);
testSame(options, code);
}
public void testFoldLocals4() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = "/** @constructor */\n"
+ "function InternalWidget(){this.x = 1;}"
+ "InternalWidget.prototype.internalGo = function (){this.x = 2};"
+ "new InternalWidget().internalGo();";
testSame(options, code);
options.computeFunctionSideEffects = true;
String optimized = ""
+ "function InternalWidget(){this.x = 1;}"
+ "InternalWidget.prototype.internalGo = function (){this.x = 2};";
test(options, code, optimized);
}
public void testFoldLocals5() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = ""
+ "function fn(){var a={};a.x={};return a}"
+ "fn().x.y = 1;";
// "fn" returns a unescaped local object, we should be able to fold it,
// but we don't currently.
String result = ""
+ "function fn(){var a={x:{}};return a}"
+ "fn().x.y = 1;";
test(options, code, result);
options.computeFunctionSideEffects = true;
test(options, code, result);
}
public void testFoldLocals6() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = ""
+ "function fn(){return {}}"
+ "fn().x.y = 1;";
testSame(options, code);
options.computeFunctionSideEffects = true;
testSame(options, code);
}
public void testFoldLocals7() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = ""
+ "function InternalWidget(){return [];}"
+ "Array.prototype.internalGo = function (){this.x = 2};"
+ "InternalWidget().internalGo();";
testSame(options, code);
options.computeFunctionSideEffects = true;
String optimized = ""
+ "function InternalWidget(){return [];}"
+ "Array.prototype.internalGo = function (){this.x = 2};";
test(options, code, optimized);
}
public void testVarDeclarationsIntoFor() {
CompilerOptions options = createCompilerOptions();
options.collapseVariableDeclarations = false;
String code = "var a = 1; for (var b = 2; ;) {}";
testSame(options, code);
options.collapseVariableDeclarations = false;
test(options, code, "for (var a = 1, b = 2; ;) {}");
}
public void testExploitAssigns() {
CompilerOptions options = createCompilerOptions();
options.collapseVariableDeclarations = false;
String code = "a = 1; b = a; c = b";
testSame(options, code);
options.collapseVariableDeclarations = true;
test(options, code, "c=b=a=1");
}
public void testRecoverOnBadExterns() throws Exception {
// This test is for a bug in a very narrow set of circumstances:
// 1) externs validation has to be off.
// 2) aliasExternals has to be on.
// 3) The user has to reference a "normal" variable in externs.
// This case is handled at checking time by injecting a
// synthetic extern variable, and adding a "@suppress {duplicate}" to
// the normal code at compile time. But optimizations may remove that
// annotation, so we need to make sure that the variable declarations
// are de-duped before that happens.
CompilerOptions options = createCompilerOptions();
options.aliasExternals = true;
externs = new JSSourceFile[] {
JSSourceFile.fromCode("externs", "extern.foo")
};
test(options,
"var extern; " +
"function f() { return extern + extern + extern + extern; }",
"var extern; " +
"function f() { return extern + extern + extern + extern; }",
VarCheck.UNDEFINED_EXTERN_VAR_ERROR);
}
public void testDuplicateVariablesInExterns() {
CompilerOptions options = createCompilerOptions();
options.checkSymbols = true;
externs = new JSSourceFile[] {
JSSourceFile.fromCode("externs",
"var externs = {}; /** @suppress {duplicate} */ var externs = {};")
};
testSame(options, "");
}
public void testLanguageMode() {
CompilerOptions options = createCompilerOptions();
options.setLanguageIn(LanguageMode.ECMASCRIPT3);
String code = "var a = {get f(){}}";
Compiler compiler = compile(options, code);
checkUnexpectedErrorsOrWarnings(compiler, 1);
assertEquals(
"JSC_PARSE_ERROR. Parse error. " +
"getters are not supported in Internet Explorer " +
"at i0 line 1 : 0",
compiler.getErrors()[0].toString());
options.setLanguageIn(LanguageMode.ECMASCRIPT5);
testSame(options, code);
options.setLanguageIn(LanguageMode.ECMASCRIPT5_STRICT);
testSame(options, code);
}
public void testLanguageMode2() {
CompilerOptions options = createCompilerOptions();
options.setLanguageIn(LanguageMode.ECMASCRIPT3);
options.setWarningLevel(DiagnosticGroups.ES5_STRICT, CheckLevel.OFF);
String code = "var a = 2; delete a;";
testSame(options, code);
options.setLanguageIn(LanguageMode.ECMASCRIPT5);
testSame(options, code);
options.setLanguageIn(LanguageMode.ECMASCRIPT5_STRICT);
test(options,
code,
code,
StrictModeCheck.DELETE_VARIABLE);
}
public void testIssue598() {
CompilerOptions options = createCompilerOptions();
options.setLanguageIn(LanguageMode.ECMASCRIPT5_STRICT);
WarningLevel.VERBOSE.setOptionsForWarningLevel(options);
options.setLanguageIn(LanguageMode.ECMASCRIPT5);
String code =
"'use strict';\n" +
"function App() {}\n" +
"App.prototype = {\n" +
" get appData() { return this.appData_; },\n" +
" set appData(data) { this.appData_ = data; }\n" +
"};";
Compiler compiler = compile(options, code);
testSame(options, code);
}
public void testCoaleseVariables() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = false;
options.coalesceVariableNames = true;
String code =
"function f(a) {" +
" if (a) {" +
" return a;" +
" } else {" +
" var b = a;" +
" return b;" +
" }" +
" return a;" +
"}";
String expected =
"function f(a) {" +
" if (a) {" +
" return a;" +
" } else {" +
" a = a;" +
" return a;" +
" }" +
" return a;" +
"}";
test(options, code, expected);
options.foldConstants = true;
options.coalesceVariableNames = false;
code =
"function f(a) {" +
" if (a) {" +
" return a;" +
" } else {" +
" var b = a;" +
" return b;" +
" }" +
" return a;" +
"}";
expected =
"function f(a) {" +
" if (!a) {" +
" var b = a;" +
" return b;" +
" }" +
" return a;" +
"}";
test(options, code, expected);
options.foldConstants = true;
options.coalesceVariableNames = true;
expected =
"function f(a) {" +
" return a;" +
"}";
test(options, code, expected);
}
public void testLateStatementFusion() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
test(options,
"while(a){a();if(b){b();b()}}",
"for(;a;)a(),b&&(b(),b())");
}
public void testLateConstantReordering() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
test(options,
"if (x < 1 || x > 1 || 1 < x || 1 > x) { alert(x) }",
" (1 > x || 1 < x || 1 < x || 1 > x) && alert(x) ");
}
public void testsyntheticBlockOnDeadAssignments() {
CompilerOptions options = createCompilerOptions();
options.deadAssignmentElimination = true;
options.removeUnusedVars = true;
options.syntheticBlockStartMarker = "START";
options.syntheticBlockEndMarker = "END";
test(options, "var x; x = 1; START(); x = 1;END();x()",
"var x; x = 1;{START();{x = 1}END()}x()");
}
public void testBug4152835() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
options.syntheticBlockStartMarker = "START";
options.syntheticBlockEndMarker = "END";
test(options, "START();END()", "{START();{}END()}");
}
public void testBug5786871() {
CompilerOptions options = createCompilerOptions();
options.ideMode = true;
test(options, "function () {}", RhinoErrorReporter.PARSE_ERROR);
}
public void testIssue378() {
CompilerOptions options = createCompilerOptions();
options.inlineVariables = true;
options.flowSensitiveInlineVariables = true;
testSame(options, "function f(c) {var f = c; arguments[0] = this;" +
" f.apply(this, arguments); return this;}");
}
public void testIssue550() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.SIMPLE_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
options.foldConstants = true;
options.inlineVariables = true;
options.flowSensitiveInlineVariables = true;
test(options,
"function f(h) {\n" +
" var a = h;\n" +
" a = a + 'x';\n" +
" a = a + 'y';\n" +
" return a;\n" +
"}",
"function f(a) {return a + 'xy'}");
}
public void testIssue284() {
CompilerOptions options = createCompilerOptions();
options.smartNameRemoval = true;
test(options,
"var goog = {};" +
"goog.inherits = function(x, y) {};" +
"var ns = {};" +
"/** @constructor */" +
"ns.PageSelectionModel = function() {};" +
"/** @constructor */" +
"ns.PageSelectionModel.FooEvent = function() {};" +
"/** @constructor */" +
"ns.PageSelectionModel.SelectEvent = function() {};" +
"goog.inherits(ns.PageSelectionModel.ChangeEvent," +
" ns.PageSelectionModel.FooEvent);",
"");
}
public void testCodingConvention() {
Compiler compiler = new Compiler();
compiler.initOptions(new CompilerOptions());
assertEquals(
compiler.getCodingConvention().getClass().toString(),
ClosureCodingConvention.class.toString());
}
public void testJQueryStringSplitLoops() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
test(options,
"var x=['1','2','3','4','5','6','7']",
"var x='1,2,3,4,5,6,7'.split(',')");
options = createCompilerOptions();
options.foldConstants = true;
options.computeFunctionSideEffects = false;
options.removeUnusedVars = true;
// If we do splits too early, it would add a sideeffect to x.
test(options,
"var x=['1','2','3','4','5','6','7']",
"");
}
public void testAlwaysRunSafetyCheck() {
CompilerOptions options = createCompilerOptions();
options.checkSymbols = false;
options.customPasses = ArrayListMultimap.create();
options.customPasses.put(
CustomPassExecutionTime.BEFORE_OPTIMIZATIONS,
new CompilerPass() {
@Override public void process(Node externs, Node root) {
Node var = root.getLastChild().getFirstChild();
assertEquals(Token.VAR, var.getType());
var.detachFromParent();
}
});
try {
test(options,
"var x = 3; function f() { return x + z; }",
"function f() { return x + z; }");
fail("Expected runtime exception");
} catch (RuntimeException e) {
assertTrue(e.getMessage().indexOf("Unexpected variable x") != -1);
}
}
public void testSuppressEs5StrictWarning() {
CompilerOptions options = createCompilerOptions();
options.setWarningLevel(DiagnosticGroups.ES5_STRICT, CheckLevel.WARNING);
testSame(options,
"/** @suppress{es5Strict} */\n" +
"function f() { var arguments; }");
}
public void testCheckProvidesWarning() {
CompilerOptions options = createCompilerOptions();
options.setWarningLevel(DiagnosticGroups.CHECK_PROVIDES, CheckLevel.WARNING);
options.setCheckProvides(CheckLevel.WARNING);
test(options,
"/** @constructor */\n" +
"function f() { var arguments; }",
DiagnosticType.warning("JSC_MISSING_PROVIDE", "missing goog.provide(''{0}'')"));
}
public void testSuppressCheckProvidesWarning() {
CompilerOptions options = createCompilerOptions();
options.setWarningLevel(DiagnosticGroups.CHECK_PROVIDES, CheckLevel.WARNING);
options.setCheckProvides(CheckLevel.WARNING);
testSame(options,
"/** @constructor\n" +
" * @suppress{checkProvides} */\n" +
"function f() { var arguments; }");
}
public void testRenamePrefixNamespace() {
String code =
"var x = {}; x.FOO = 5; x.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.collapseProperties = true;
options.renamePrefixNamespace = "_";
test(options, code, "_.x$FOO = 5; _.x$bar = 3;");
}
public void testRenamePrefixNamespaceActivatesMoveFunctionDeclarations() {
CompilerOptions options = createCompilerOptions();
String code = "var x = f; function f() { return 3; }";
testSame(options, code);
assertFalse(options.moveFunctionDeclarations);
options.renamePrefixNamespace = "_";
test(options, code, "_.f = function() { return 3; }; _.x = _.f;");
}
public void testBrokenNameSpace() {
CompilerOptions options = createCompilerOptions();
String code = "var goog; goog.provide('i.am.on.a.Horse');" +
"i.am.on.a.Horse = function() {};" +
"i.am.on.a.Horse.prototype.x = function() {};" +
"i.am.on.a.Boat.prototype.y = function() {}";
options.closurePass = true;
options.collapseProperties = true;
options.smartNameRemoval = true;
test(options, code, "");
}
public void testNamelessParameter() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.ADVANCED_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
String code =
"var impl_0;" +
"$load($init());" +
"function $load(){" +
" window['f'] = impl_0;" +
"}" +
"function $init() {" +
" impl_0 = {};" +
"}";
String result =
"window.f = {};";
test(options, code, result);
}
public void testHiddenSideEffect() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.ADVANCED_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
options.setAliasExternals(true);
String code =
"window.offsetWidth;";
String result =
"window.offsetWidth;";
test(options, code, result);
}
public void testNegativeZero() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.ADVANCED_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
test(options,
"function bar(x) { return x; }\n" +
"function foo(x) { print(x / bar(0));\n" +
" print(x / bar(-0)); }\n" +
"foo(3);",
"print(3/0);print(3/-0);");
}
public void testSingletonGetter1() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.ADVANCED_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
options.setCodingConvention(new ClosureCodingConvention());
test(options,
"/** @const */\n" +
"var goog = goog || {};\n" +
"goog.addSingletonGetter = function(ctor) {\n" +
" ctor.getInstance = function() {\n" +
" return ctor.instance_ || (ctor.instance_ = new ctor());\n" +
" };\n" +
"};" +
"function Foo() {}\n" +
"goog.addSingletonGetter(Foo);" +
"Foo.prototype.bar = 1;" +
"function Bar() {}\n" +
"goog.addSingletonGetter(Bar);" +
"Bar.prototype.bar = 1;",
"");
}
public void testIncompleteFunction() {
CompilerOptions options = createCompilerOptions();
options.ideMode = true;
DiagnosticType[] warnings = new DiagnosticType[]{
RhinoErrorReporter.PARSE_ERROR,
RhinoErrorReporter.PARSE_ERROR,
RhinoErrorReporter.PARSE_ERROR,
RhinoErrorReporter.PARSE_ERROR};
test(options,
new String[] { "var foo = {bar: function(e) }" },
new String[] { "var foo = {bar: function(e){}};" },
warnings
);
}
private void testSame(CompilerOptions options, String original) {
testSame(options, new String[] { original });
}
private void testSame(CompilerOptions options, String[] original) {
test(options, original, original);
}
/**
* Asserts that when compiling with the given compiler options,
* {@code original} is transformed into {@code compiled}.
*/
private void test(CompilerOptions options,
String original, String compiled) {
test(options, new String[] { original }, new String[] { compiled });
}
/**
* Asserts that when compiling with the given compiler options,
* {@code original} is transformed into {@code compiled}.
*/
private void test(CompilerOptions options,
String[] original, String[] compiled) {
Compiler compiler = compile(options, original);
assertEquals("Expected no warnings or errors\n" +
"Errors: \n" + Joiner.on("\n").join(compiler.getErrors()) +
"Warnings: \n" + Joiner.on("\n").join(compiler.getWarnings()),
0, compiler.getErrors().length + compiler.getWarnings().length);
Node root = compiler.getRoot().getLastChild();
Node expectedRoot = parse(compiled, options);
String explanation = expectedRoot.checkTreeEquals(root);
assertNull("\nExpected: " + compiler.toSource(expectedRoot) +
"\nResult: " + compiler.toSource(root) +
"\n" + explanation, explanation);
}
/**
* Asserts that when compiling with the given compiler options,
* there is an error or warning.
*/
private void test(CompilerOptions options,
String original, DiagnosticType warning) {
test(options, new String[] { original }, warning);
}
private void test(CompilerOptions options,
String original, String compiled, DiagnosticType warning) {
test(options, new String[] { original }, new String[] { compiled },
warning);
}
private void test(CompilerOptions options,
String[] original, DiagnosticType warning) {
test(options, original, null, warning);
}
/**
* Asserts that when compiling with the given compiler options,
* there is an error or warning.
*/
private void test(CompilerOptions options,
String[] original, String[] compiled, DiagnosticType warning) {
Compiler compiler = compile(options, original);
checkUnexpectedErrorsOrWarnings(compiler, 1);
assertEquals("Expected exactly one warning or error",
1, compiler.getErrors().length + compiler.getWarnings().length);
if (compiler.getErrors().length > 0) {
assertEquals(warning, compiler.getErrors()[0].getType());
} else {
assertEquals(warning, compiler.getWarnings()[0].getType());
}
if (compiled != null) {
Node root = compiler.getRoot().getLastChild();
Node expectedRoot = parse(compiled, options);
String explanation = expectedRoot.checkTreeEquals(root);
assertNull("\nExpected: " + compiler.toSource(expectedRoot) +
"\nResult: " + compiler.toSource(root) +
"\n" + explanation, explanation);
}
}
/**
* Asserts that when compiling with the given compiler options,
* there is an error or warning.
*/
private void test(CompilerOptions options,
String[] original, String[] compiled, DiagnosticType[] warnings) {
Compiler compiler = compile(options, original);
checkUnexpectedErrorsOrWarnings(compiler, warnings.length);
if (compiled != null) {
Node root = compiler.getRoot().getLastChild();
Node expectedRoot = parse(compiled, options);
String explanation = expectedRoot.checkTreeEquals(root);
assertNull("\nExpected: " + compiler.toSource(expectedRoot) +
"\nResult: " + compiler.toSource(root) +
"\n" + explanation, explanation);
}
}
private void checkUnexpectedErrorsOrWarnings(
Compiler compiler, int expected) {
int actual = compiler.getErrors().length + compiler.getWarnings().length;
if (actual != expected) {
String msg = "";
for (JSError err : compiler.getErrors()) {
msg += "Error:" + err.toString() + "\n";
}
for (JSError err : compiler.getWarnings()) {
msg += "Warning:" + err.toString() + "\n";
}
assertEquals("Unexpected warnings or errors.\n "+ msg,
expected, actual);
}
}
private Compiler compile(CompilerOptions options, String original) {
return compile(options, new String[] { original });
}
private Compiler compile(CompilerOptions options, String[] original) {
Compiler compiler = lastCompiler = new Compiler();
JSSourceFile[] inputs = new JSSourceFile[original.length];
for (int i = 0; i < original.length; i++) {
inputs[i] = JSSourceFile.fromCode("input" + i, original[i]);
}
compiler.compile(
externs, CompilerTestCase.createModuleChain(original), options);
return compiler;
}
private Node parse(String[] original, CompilerOptions options) {
Compiler compiler = new Compiler();
JSSourceFile[] inputs = new JSSourceFile[original.length];
for (int i = 0; i < inputs.length; i++) {
inputs[i] = JSSourceFile.fromCode("input" + i, original[i]);
}
compiler.init(externs, inputs, options);
checkUnexpectedErrorsOrWarnings(compiler, 0);
Node all = compiler.parseInputs();
checkUnexpectedErrorsOrWarnings(compiler, 0);
Node n = all.getLastChild();
Node externs = all.getFirstChild();
(new CreateSyntheticBlocks(
compiler, "synStart", "synEnd")).process(externs, n);
(new Normalize(compiler, false)).process(externs, n);
(MakeDeclaredNamesUnique.getContextualRenameInverter(compiler)).process(
externs, n);
(new Denormalize(compiler)).process(externs, n);
return n;
}
/** Creates a CompilerOptions object with google coding conventions. */
private CompilerOptions createCompilerOptions() {
CompilerOptions options = new CompilerOptions();
options.setCodingConvention(new GoogleCodingConvention());
return options;
}
} | // You are a professional Java test case writer, please create a test case named `testSingletonGetter1` for the issue `Closure-668`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-668
//
// ## Issue-Title:
// goog.addSingletonGetter prevents unused class removal
//
// ## Issue-Description:
// **What steps will reproduce the problem?**
//
// // ==ClosureCompiler==
// // @compilation\_level ADVANCED\_OPTIMIZATIONS
// // @output\_file\_name default.js
// // @use\_closure\_library true
// // @formatting pretty\_print,print\_input\_delimiter
// // @warning\_level VERBOSE
// // @debug true
// // ==/ClosureCompiler==
//
// goog.provide('foo');
//
// var foo = function() { this.values = []; };
// goog.addSingletonGetter(foo);
//
// foo.prototype.add = function(value) {this.values.push(value)};
//
//
// **What is the expected output? What do you see instead?**
//
// Expect: The code is completely removed.
//
// Instead:
//
// (function($ctor$$) {
// $ctor$$.$getInstance$ = function $$ctor$$$$getInstance$$() {
// return $ctor$$.$instance\_$ || ($ctor$$.$instance\_$ = new $ctor$$)
// }
// })(function() {
// });
//
//
// What version of the product are you using? On what operating system?
//
// http://closure-compiler.appspot.com on Feb 28, 2012
//
// Please provide any additional information below.
//
//
public void testSingletonGetter1() {
| 1,956 | 36 | 1,936 | test/com/google/javascript/jscomp/IntegrationTest.java | test | ```markdown
## Issue-ID: Closure-668
## Issue-Title:
goog.addSingletonGetter prevents unused class removal
## Issue-Description:
**What steps will reproduce the problem?**
// ==ClosureCompiler==
// @compilation\_level ADVANCED\_OPTIMIZATIONS
// @output\_file\_name default.js
// @use\_closure\_library true
// @formatting pretty\_print,print\_input\_delimiter
// @warning\_level VERBOSE
// @debug true
// ==/ClosureCompiler==
goog.provide('foo');
var foo = function() { this.values = []; };
goog.addSingletonGetter(foo);
foo.prototype.add = function(value) {this.values.push(value)};
**What is the expected output? What do you see instead?**
Expect: The code is completely removed.
Instead:
(function($ctor$$) {
$ctor$$.$getInstance$ = function $$ctor$$$$getInstance$$() {
return $ctor$$.$instance\_$ || ($ctor$$.$instance\_$ = new $ctor$$)
}
})(function() {
});
What version of the product are you using? On what operating system?
http://closure-compiler.appspot.com on Feb 28, 2012
Please provide any additional information below.
```
You are a professional Java test case writer, please create a test case named `testSingletonGetter1` for the issue `Closure-668`, utilizing the provided issue report information and the following function signature.
```java
public void testSingletonGetter1() {
```
| 1,936 | [
"com.google.javascript.jscomp.InlineVariables"
] | 77aa0aed1809e86e43b5da3f38514fdedf9bb68b26b7dce6ed9acbaa650f74eb | public void testSingletonGetter1() | // You are a professional Java test case writer, please create a test case named `testSingletonGetter1` for the issue `Closure-668`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-668
//
// ## Issue-Title:
// goog.addSingletonGetter prevents unused class removal
//
// ## Issue-Description:
// **What steps will reproduce the problem?**
//
// // ==ClosureCompiler==
// // @compilation\_level ADVANCED\_OPTIMIZATIONS
// // @output\_file\_name default.js
// // @use\_closure\_library true
// // @formatting pretty\_print,print\_input\_delimiter
// // @warning\_level VERBOSE
// // @debug true
// // ==/ClosureCompiler==
//
// goog.provide('foo');
//
// var foo = function() { this.values = []; };
// goog.addSingletonGetter(foo);
//
// foo.prototype.add = function(value) {this.values.push(value)};
//
//
// **What is the expected output? What do you see instead?**
//
// Expect: The code is completely removed.
//
// Instead:
//
// (function($ctor$$) {
// $ctor$$.$getInstance$ = function $$ctor$$$$getInstance$$() {
// return $ctor$$.$instance\_$ || ($ctor$$.$instance\_$ = new $ctor$$)
// }
// })(function() {
// });
//
//
// What version of the product are you using? On what operating system?
//
// http://closure-compiler.appspot.com on Feb 28, 2012
//
// Please provide any additional information below.
//
//
| Closure | /*
* Copyright 2009 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import com.google.common.base.Joiner;
import com.google.common.collect.ArrayListMultimap;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.Sets;
import com.google.javascript.jscomp.CompilerOptions.LanguageMode;
import com.google.javascript.rhino.Node;
import com.google.javascript.rhino.Token;
import junit.framework.TestCase;
/**
* Tests for {@link PassFactory}.
*
* @author [email protected] (Nick Santos)
*/
public class IntegrationTest extends TestCase {
/** Externs for the test */
private final JSSourceFile[] DEFAULT_EXTERNS = new JSSourceFile[] {
JSSourceFile.fromCode("externs",
"var arguments;\n"
+ "/** @constructor */ function Window() {}\n"
+ "/** @type {string} */ Window.prototype.name;\n"
+ "/** @type {string} */ Window.prototype.offsetWidth;\n"
+ "/** @type {Window} */ var window;\n"
+ "/** @nosideeffects */ function noSideEffects() {}\n"
+ "/** @constructor\n * @nosideeffects */ function Widget() {}\n"
+ "/** @modifies {this} */ Widget.prototype.go = function() {};\n"
+ "/** @return {string} */ var widgetToken = function() {};\n")
};
private JSSourceFile[] externs = DEFAULT_EXTERNS;
private static final String CLOSURE_BOILERPLATE =
"/** @define {boolean} */ var COMPILED = false; var goog = {};" +
"goog.exportSymbol = function() {};";
private static final String CLOSURE_COMPILED =
"var COMPILED = true; var goog$exportSymbol = function() {};";
// The most recently used compiler.
private Compiler lastCompiler;
@Override
public void setUp() {
externs = DEFAULT_EXTERNS;
lastCompiler = null;
}
public void testBug1949424() {
CompilerOptions options = createCompilerOptions();
options.collapseProperties = true;
options.closurePass = true;
test(options, CLOSURE_BOILERPLATE + "goog.provide('FOO'); FOO.bar = 3;",
CLOSURE_COMPILED + "var FOO$bar = 3;");
}
public void testBug1949424_v2() {
CompilerOptions options = createCompilerOptions();
options.collapseProperties = true;
options.closurePass = true;
test(options, CLOSURE_BOILERPLATE + "goog.provide('FOO.BAR'); FOO.BAR = 3;",
CLOSURE_COMPILED + "var FOO$BAR = 3;");
}
public void testBug1956277() {
CompilerOptions options = createCompilerOptions();
options.collapseProperties = true;
options.inlineVariables = true;
test(options, "var CONST = {}; CONST.bar = null;" +
"function f(url) { CONST.bar = url; }",
"var CONST$bar = null; function f(url) { CONST$bar = url; }");
}
public void testBug1962380() {
CompilerOptions options = createCompilerOptions();
options.collapseProperties = true;
options.inlineVariables = true;
options.generateExports = true;
test(options,
CLOSURE_BOILERPLATE + "/** @export */ goog.CONSTANT = 1;" +
"var x = goog.CONSTANT;",
"(function() {})('goog.CONSTANT', 1);" +
"var x = 1;");
}
public void testBug2410122() {
CompilerOptions options = createCompilerOptions();
options.generateExports = true;
options.closurePass = true;
test(options,
"var goog = {};" +
"function F() {}" +
"/** @export */ function G() { goog.base(this); } " +
"goog.inherits(G, F);",
"var goog = {};" +
"function F() {}" +
"function G() { F.call(this); } " +
"goog.inherits(G, F); goog.exportSymbol('G', G);");
}
public void testIssue90() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
options.inlineVariables = true;
options.removeDeadCode = true;
test(options,
"var x; x && alert(1);",
"");
}
public void testClosurePassOff() {
CompilerOptions options = createCompilerOptions();
options.closurePass = false;
testSame(
options,
"var goog = {}; goog.require = function(x) {}; goog.require('foo');");
testSame(
options,
"var goog = {}; goog.getCssName = function(x) {};" +
"goog.getCssName('foo');");
}
public void testClosurePassOn() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
test(
options,
"var goog = {}; goog.require = function(x) {}; goog.require('foo');",
ProcessClosurePrimitives.MISSING_PROVIDE_ERROR);
test(
options,
"/** @define {boolean} */ var COMPILED = false;" +
"var goog = {}; goog.getCssName = function(x) {};" +
"goog.getCssName('foo');",
"var COMPILED = true;" +
"var goog = {}; goog.getCssName = function(x) {};" +
"'foo';");
}
public void testCssNameCheck() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.checkMissingGetCssNameLevel = CheckLevel.ERROR;
options.checkMissingGetCssNameBlacklist = "foo";
test(options, "var x = 'foo';",
CheckMissingGetCssName.MISSING_GETCSSNAME);
}
public void testBug2592659() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.checkTypes = true;
options.checkMissingGetCssNameLevel = CheckLevel.WARNING;
options.checkMissingGetCssNameBlacklist = "foo";
test(options,
"var goog = {};\n" +
"/**\n" +
" * @param {string} className\n" +
" * @param {string=} opt_modifier\n" +
" * @return {string}\n" +
"*/\n" +
"goog.getCssName = function(className, opt_modifier) {}\n" +
"var x = goog.getCssName(123, 'a');",
TypeValidator.TYPE_MISMATCH_WARNING);
}
public void testTypedefBeforeOwner1() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
test(options,
"goog.provide('foo.Bar.Type');\n" +
"goog.provide('foo.Bar');\n" +
"/** @typedef {number} */ foo.Bar.Type;\n" +
"foo.Bar = function() {};",
"var foo = {}; foo.Bar.Type; foo.Bar = function() {};");
}
public void testTypedefBeforeOwner2() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.collapseProperties = true;
test(options,
"goog.provide('foo.Bar.Type');\n" +
"goog.provide('foo.Bar');\n" +
"/** @typedef {number} */ foo.Bar.Type;\n" +
"foo.Bar = function() {};",
"var foo$Bar$Type; var foo$Bar = function() {};");
}
public void testExportedNames() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.variableRenaming = VariableRenamingPolicy.ALL;
test(options,
"/** @define {boolean} */ var COMPILED = false;" +
"var goog = {}; goog.exportSymbol('b', goog);",
"var a = true; var c = {}; c.exportSymbol('b', c);");
test(options,
"/** @define {boolean} */ var COMPILED = false;" +
"var goog = {}; goog.exportSymbol('a', goog);",
"var b = true; var c = {}; c.exportSymbol('a', c);");
}
public void testCheckGlobalThisOn() {
CompilerOptions options = createCompilerOptions();
options.checkSuspiciousCode = true;
options.checkGlobalThisLevel = CheckLevel.ERROR;
test(options, "function f() { this.y = 3; }", CheckGlobalThis.GLOBAL_THIS);
}
public void testSusiciousCodeOff() {
CompilerOptions options = createCompilerOptions();
options.checkSuspiciousCode = false;
options.checkGlobalThisLevel = CheckLevel.ERROR;
test(options, "function f() { this.y = 3; }", CheckGlobalThis.GLOBAL_THIS);
}
public void testCheckGlobalThisOff() {
CompilerOptions options = createCompilerOptions();
options.checkSuspiciousCode = true;
options.checkGlobalThisLevel = CheckLevel.OFF;
testSame(options, "function f() { this.y = 3; }");
}
public void testCheckRequiresAndCheckProvidesOff() {
testSame(createCompilerOptions(), new String[] {
"/** @constructor */ function Foo() {}",
"new Foo();"
});
}
public void testCheckRequiresOn() {
CompilerOptions options = createCompilerOptions();
options.checkRequires = CheckLevel.ERROR;
test(options, new String[] {
"/** @constructor */ function Foo() {}",
"new Foo();"
}, CheckRequiresForConstructors.MISSING_REQUIRE_WARNING);
}
public void testCheckProvidesOn() {
CompilerOptions options = createCompilerOptions();
options.checkProvides = CheckLevel.ERROR;
test(options, new String[] {
"/** @constructor */ function Foo() {}",
"new Foo();"
}, CheckProvides.MISSING_PROVIDE_WARNING);
}
public void testGenerateExportsOff() {
testSame(createCompilerOptions(), "/** @export */ function f() {}");
}
public void testGenerateExportsOn() {
CompilerOptions options = createCompilerOptions();
options.generateExports = true;
test(options, "/** @export */ function f() {}",
"/** @export */ function f() {} goog.exportSymbol('f', f);");
}
public void testExportTestFunctionsOff() {
testSame(createCompilerOptions(), "function testFoo() {}");
}
public void testExportTestFunctionsOn() {
CompilerOptions options = createCompilerOptions();
options.exportTestFunctions = true;
test(options, "function testFoo() {}",
"/** @export */ function testFoo() {}" +
"goog.exportSymbol('testFoo', testFoo);");
}
public void testCheckSymbolsOff() {
CompilerOptions options = createCompilerOptions();
testSame(options, "x = 3;");
}
public void testCheckSymbolsOn() {
CompilerOptions options = createCompilerOptions();
options.checkSymbols = true;
test(options, "x = 3;", VarCheck.UNDEFINED_VAR_ERROR);
}
public void testCheckReferencesOff() {
CompilerOptions options = createCompilerOptions();
testSame(options, "x = 3; var x = 5;");
}
public void testCheckReferencesOn() {
CompilerOptions options = createCompilerOptions();
options.aggressiveVarCheck = CheckLevel.ERROR;
test(options, "x = 3; var x = 5;",
VariableReferenceCheck.UNDECLARED_REFERENCE);
}
public void testInferTypes() {
CompilerOptions options = createCompilerOptions();
options.inferTypes = true;
options.checkTypes = false;
options.closurePass = true;
test(options,
CLOSURE_BOILERPLATE +
"goog.provide('Foo'); /** @enum */ Foo = {a: 3};",
TypeCheck.ENUM_NOT_CONSTANT);
assertTrue(lastCompiler.getErrorManager().getTypedPercent() == 0);
// This does not generate a warning.
test(options, "/** @type {number} */ var n = window.name;",
"var n = window.name;");
assertTrue(lastCompiler.getErrorManager().getTypedPercent() == 0);
}
public void testTypeCheckAndInference() {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
test(options, "/** @type {number} */ var n = window.name;",
TypeValidator.TYPE_MISMATCH_WARNING);
assertTrue(lastCompiler.getErrorManager().getTypedPercent() > 0);
}
public void testTypeNameParser() {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
test(options, "/** @type {n} */ var n = window.name;",
RhinoErrorReporter.TYPE_PARSE_ERROR);
}
// This tests that the TypedScopeCreator is memoized so that it only creates a
// Scope object once for each scope. If, when type inference requests a scope,
// it creates a new one, then multiple JSType objects end up getting created
// for the same local type, and ambiguate will rename the last statement to
// o.a(o.a, o.a), which is bad.
public void testMemoizedTypedScopeCreator() {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
options.ambiguateProperties = true;
options.propertyRenaming = PropertyRenamingPolicy.ALL_UNQUOTED;
test(options, "function someTest() {\n"
+ " /** @constructor */\n"
+ " function Foo() { this.instProp = 3; }\n"
+ " Foo.prototype.protoProp = function(a, b) {};\n"
+ " /** @constructor\n @extends Foo */\n"
+ " function Bar() {}\n"
+ " goog.inherits(Bar, Foo);\n"
+ " var o = new Bar();\n"
+ " o.protoProp(o.protoProp, o.instProp);\n"
+ "}",
"function someTest() {\n"
+ " function Foo() { this.b = 3; }\n"
+ " Foo.prototype.a = function(a, b) {};\n"
+ " function Bar() {}\n"
+ " goog.c(Bar, Foo);\n"
+ " var o = new Bar();\n"
+ " o.a(o.a, o.b);\n"
+ "}");
}
public void testCheckTypes() {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
test(options, "var x = x || {}; x.f = function() {}; x.f(3);",
TypeCheck.WRONG_ARGUMENT_COUNT);
}
public void testReplaceCssNames() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.gatherCssNames = true;
test(options, "/** @define {boolean} */\n"
+ "var COMPILED = false;\n"
+ "goog.setCssNameMapping({'foo':'bar'});\n"
+ "function getCss() {\n"
+ " return goog.getCssName('foo');\n"
+ "}",
"var COMPILED = true;\n"
+ "function getCss() {\n"
+ " return \"bar\";"
+ "}");
assertEquals(
ImmutableMap.of("foo", new Integer(1)),
lastCompiler.getPassConfig().getIntermediateState().cssNames);
}
public void testRemoveClosureAsserts() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
testSame(options,
"var goog = {};"
+ "goog.asserts.assert(goog);");
options.removeClosureAsserts = true;
test(options,
"var goog = {};"
+ "goog.asserts.assert(goog);",
"var goog = {};");
}
public void testDeprecation() {
String code = "/** @deprecated */ function f() { } function g() { f(); }";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.setWarningLevel(DiagnosticGroups.DEPRECATED, CheckLevel.ERROR);
testSame(options, code);
options.checkTypes = true;
test(options, code, CheckAccessControls.DEPRECATED_NAME);
}
public void testVisibility() {
String[] code = {
"/** @private */ function f() { }",
"function g() { f(); }"
};
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.setWarningLevel(DiagnosticGroups.VISIBILITY, CheckLevel.ERROR);
testSame(options, code);
options.checkTypes = true;
test(options, code, CheckAccessControls.BAD_PRIVATE_GLOBAL_ACCESS);
}
public void testUnreachableCode() {
String code = "function f() { return \n 3; }";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.checkUnreachableCode = CheckLevel.ERROR;
test(options, code, CheckUnreachableCode.UNREACHABLE_CODE);
}
public void testMissingReturn() {
String code =
"/** @return {number} */ function f() { if (f) { return 3; } }";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.checkMissingReturn = CheckLevel.ERROR;
testSame(options, code);
options.checkTypes = true;
test(options, code, CheckMissingReturn.MISSING_RETURN_STATEMENT);
}
public void testIdGenerators() {
String code = "function f() {} f('id');";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.idGenerators = Sets.newHashSet("f");
test(options, code, "function f() {} 'a';");
}
public void testOptimizeArgumentsArray() {
String code = "function f() { return arguments[0]; }";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.optimizeArgumentsArray = true;
String argName = "JSCompiler_OptimizeArgumentsArray_p0";
test(options, code,
"function f(" + argName + ") { return " + argName + "; }");
}
public void testOptimizeParameters() {
String code = "function f(a) { return a; } f(true);";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.optimizeParameters = true;
test(options, code, "function f() { var a = true; return a;} f();");
}
public void testOptimizeReturns() {
String code = "function f(a) { return a; } f(true);";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.optimizeReturns = true;
test(options, code, "function f(a) {return;} f(true);");
}
public void testRemoveAbstractMethods() {
String code = CLOSURE_BOILERPLATE +
"var x = {}; x.foo = goog.abstractMethod; x.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.closurePass = true;
options.collapseProperties = true;
test(options, code, CLOSURE_COMPILED + " var x$bar = 3;");
}
public void testCollapseProperties1() {
String code =
"var x = {}; x.FOO = 5; x.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.collapseProperties = true;
test(options, code, "var x$FOO = 5; var x$bar = 3;");
}
public void testCollapseProperties2() {
String code =
"var x = {}; x.FOO = 5; x.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.collapseProperties = true;
options.collapseObjectLiterals = true;
test(options, code, "var x$FOO = 5; var x$bar = 3;");
}
public void testCollapseObjectLiteral1() {
// Verify collapseObjectLiterals does nothing in global scope
String code = "var x = {}; x.FOO = 5; x.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.collapseObjectLiterals = true;
testSame(options, code);
}
public void testCollapseObjectLiteral2() {
String code =
"function f() {var x = {}; x.FOO = 5; x.bar = 3;}";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.collapseObjectLiterals = true;
test(options, code,
"function f(){" +
"var JSCompiler_object_inline_FOO_0;" +
"var JSCompiler_object_inline_bar_1;" +
"JSCompiler_object_inline_FOO_0=5;" +
"JSCompiler_object_inline_bar_1=3}");
}
public void testTightenTypesWithoutTypeCheck() {
CompilerOptions options = createCompilerOptions();
options.tightenTypes = true;
test(options, "", DefaultPassConfig.TIGHTEN_TYPES_WITHOUT_TYPE_CHECK);
}
public void testDisambiguateProperties() {
String code =
"/** @constructor */ function Foo(){} Foo.prototype.bar = 3;" +
"/** @constructor */ function Baz(){} Baz.prototype.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.disambiguateProperties = true;
options.checkTypes = true;
test(options, code,
"function Foo(){} Foo.prototype.Foo_prototype$bar = 3;" +
"function Baz(){} Baz.prototype.Baz_prototype$bar = 3;");
}
public void testMarkPureCalls() {
String testCode = "function foo() {} foo();";
CompilerOptions options = createCompilerOptions();
options.removeDeadCode = true;
testSame(options, testCode);
options.computeFunctionSideEffects = true;
test(options, testCode, "function foo() {}");
}
public void testMarkNoSideEffects() {
String testCode = "noSideEffects();";
CompilerOptions options = createCompilerOptions();
options.removeDeadCode = true;
testSame(options, testCode);
options.markNoSideEffectCalls = true;
test(options, testCode, "");
}
public void testChainedCalls() {
CompilerOptions options = createCompilerOptions();
options.chainCalls = true;
test(
options,
"/** @constructor */ function Foo() {} " +
"Foo.prototype.bar = function() { return this; }; " +
"var f = new Foo();" +
"f.bar(); " +
"f.bar(); ",
"function Foo() {} " +
"Foo.prototype.bar = function() { return this; }; " +
"var f = new Foo();" +
"f.bar().bar();");
}
public void testExtraAnnotationNames() {
CompilerOptions options = createCompilerOptions();
options.setExtraAnnotationNames(Sets.newHashSet("TagA", "TagB"));
test(
options,
"/** @TagA */ var f = new Foo(); /** @TagB */ f.bar();",
"var f = new Foo(); f.bar();");
}
public void testDevirtualizePrototypeMethods() {
CompilerOptions options = createCompilerOptions();
options.devirtualizePrototypeMethods = true;
test(
options,
"/** @constructor */ var Foo = function() {}; " +
"Foo.prototype.bar = function() {};" +
"(new Foo()).bar();",
"var Foo = function() {};" +
"var JSCompiler_StaticMethods_bar = " +
" function(JSCompiler_StaticMethods_bar$self) {};" +
"JSCompiler_StaticMethods_bar(new Foo());");
}
public void testCheckConsts() {
CompilerOptions options = createCompilerOptions();
options.inlineConstantVars = true;
test(options, "var FOO = true; FOO = false",
ConstCheck.CONST_REASSIGNED_VALUE_ERROR);
}
public void testAllChecksOn() {
CompilerOptions options = createCompilerOptions();
options.checkSuspiciousCode = true;
options.checkControlStructures = true;
options.checkRequires = CheckLevel.ERROR;
options.checkProvides = CheckLevel.ERROR;
options.generateExports = true;
options.exportTestFunctions = true;
options.closurePass = true;
options.checkMissingGetCssNameLevel = CheckLevel.ERROR;
options.checkMissingGetCssNameBlacklist = "goog";
options.syntheticBlockStartMarker = "synStart";
options.syntheticBlockEndMarker = "synEnd";
options.checkSymbols = true;
options.aggressiveVarCheck = CheckLevel.ERROR;
options.processObjectPropertyString = true;
options.collapseProperties = true;
test(options, CLOSURE_BOILERPLATE, CLOSURE_COMPILED);
}
public void testTypeCheckingWithSyntheticBlocks() {
CompilerOptions options = createCompilerOptions();
options.syntheticBlockStartMarker = "synStart";
options.syntheticBlockEndMarker = "synEnd";
options.checkTypes = true;
// We used to have a bug where the CFG drew an
// edge straight from synStart to f(progress).
// If that happens, then progress will get type {number|undefined}.
testSame(
options,
"/** @param {number} x */ function f(x) {}" +
"function g() {" +
" synStart('foo');" +
" var progress = 1;" +
" f(progress);" +
" synEnd('foo');" +
"}");
}
public void testCompilerDoesNotBlowUpIfUndefinedSymbols() {
CompilerOptions options = createCompilerOptions();
options.checkSymbols = true;
// Disable the undefined variable check.
options.setWarningLevel(
DiagnosticGroup.forType(VarCheck.UNDEFINED_VAR_ERROR),
CheckLevel.OFF);
// The compiler used to throw an IllegalStateException on this.
testSame(options, "var x = {foo: y};");
}
// Make sure that if we change variables which are constant to have
// $$constant appended to their names, we remove that tag before
// we finish.
public void testConstantTagsMustAlwaysBeRemoved() {
CompilerOptions options = createCompilerOptions();
options.variableRenaming = VariableRenamingPolicy.LOCAL;
String originalText = "var G_GEO_UNKNOWN_ADDRESS=1;\n" +
"function foo() {" +
" var localVar = 2;\n" +
" if (G_GEO_UNKNOWN_ADDRESS == localVar) {\n" +
" alert(\"A\"); }}";
String expectedText = "var G_GEO_UNKNOWN_ADDRESS=1;" +
"function foo(){var a=2;if(G_GEO_UNKNOWN_ADDRESS==a){alert(\"A\")}}";
test(options, originalText, expectedText);
}
public void testClosurePassPreservesJsDoc() {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
options.closurePass = true;
test(options,
CLOSURE_BOILERPLATE +
"goog.provide('Foo'); /** @constructor */ Foo = function() {};" +
"var x = new Foo();",
"var COMPILED=true;var goog={};goog.exportSymbol=function(){};" +
"var Foo=function(){};var x=new Foo");
test(options,
CLOSURE_BOILERPLATE +
"goog.provide('Foo'); /** @enum */ Foo = {a: 3};",
TypeCheck.ENUM_NOT_CONSTANT);
}
public void testProvidedNamespaceIsConst() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.inlineConstantVars = true;
options.collapseProperties = true;
test(options,
"var goog = {}; goog.provide('foo'); " +
"function f() { foo = {};}",
"var foo = {}; function f() { foo = {}; }",
ConstCheck.CONST_REASSIGNED_VALUE_ERROR);
}
public void testProvidedNamespaceIsConst2() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.inlineConstantVars = true;
options.collapseProperties = true;
test(options,
"var goog = {}; goog.provide('foo.bar'); " +
"function f() { foo.bar = {};}",
"var foo$bar = {};" +
"function f() { foo$bar = {}; }",
ConstCheck.CONST_REASSIGNED_VALUE_ERROR);
}
public void testProvidedNamespaceIsConst3() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.inlineConstantVars = true;
options.collapseProperties = true;
test(options,
"var goog = {}; " +
"goog.provide('foo.bar'); goog.provide('foo.bar.baz'); " +
"/** @constructor */ foo.bar = function() {};" +
"/** @constructor */ foo.bar.baz = function() {};",
"var foo$bar = function(){};" +
"var foo$bar$baz = function(){};");
}
public void testProvidedNamespaceIsConst4() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.inlineConstantVars = true;
options.collapseProperties = true;
test(options,
"var goog = {}; goog.provide('foo.Bar'); " +
"var foo = {}; foo.Bar = {};",
"var foo = {}; var foo = {}; foo.Bar = {};");
}
public void testProvidedNamespaceIsConst5() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.inlineConstantVars = true;
options.collapseProperties = true;
test(options,
"var goog = {}; goog.provide('foo.Bar'); " +
"foo = {}; foo.Bar = {};",
"var foo = {}; foo = {}; foo.Bar = {};");
}
public void testProcessDefinesAlwaysOn() {
test(createCompilerOptions(),
"/** @define {boolean} */ var HI = true; HI = false;",
"var HI = false;false;");
}
public void testProcessDefinesAdditionalReplacements() {
CompilerOptions options = createCompilerOptions();
options.setDefineToBooleanLiteral("HI", false);
test(options,
"/** @define {boolean} */ var HI = true;",
"var HI = false;");
}
public void testReplaceMessages() {
CompilerOptions options = createCompilerOptions();
String prefix = "var goog = {}; goog.getMsg = function() {};";
testSame(options, prefix + "var MSG_HI = goog.getMsg('hi');");
options.messageBundle = new EmptyMessageBundle();
test(options,
prefix + "/** @desc xyz */ var MSG_HI = goog.getMsg('hi');",
prefix + "var MSG_HI = 'hi';");
}
public void testCheckGlobalNames() {
CompilerOptions options = createCompilerOptions();
options.checkGlobalNamesLevel = CheckLevel.ERROR;
test(options, "var x = {}; var y = x.z;",
CheckGlobalNames.UNDEFINED_NAME_WARNING);
}
public void testInlineGetters() {
CompilerOptions options = createCompilerOptions();
String code =
"function Foo() {} Foo.prototype.bar = function() { return 3; };" +
"var x = new Foo(); x.bar();";
testSame(options, code);
options.inlineGetters = true;
test(options, code,
"function Foo() {} Foo.prototype.bar = function() { return 3 };" +
"var x = new Foo(); 3;");
}
public void testInlineGettersWithAmbiguate() {
CompilerOptions options = createCompilerOptions();
String code =
"/** @constructor */" +
"function Foo() {}" +
"/** @type {number} */ Foo.prototype.field;" +
"Foo.prototype.getField = function() { return this.field; };" +
"/** @constructor */" +
"function Bar() {}" +
"/** @type {string} */ Bar.prototype.field;" +
"Bar.prototype.getField = function() { return this.field; };" +
"new Foo().getField();" +
"new Bar().getField();";
testSame(options, code);
options.inlineGetters = true;
test(options, code,
"function Foo() {}" +
"Foo.prototype.field;" +
"Foo.prototype.getField = function() { return this.field; };" +
"function Bar() {}" +
"Bar.prototype.field;" +
"Bar.prototype.getField = function() { return this.field; };" +
"new Foo().field;" +
"new Bar().field;");
options.checkTypes = true;
options.ambiguateProperties = true;
// Propagating the wrong type information may cause ambiguate properties
// to generate bad code.
testSame(options, code);
}
public void testInlineVariables() {
CompilerOptions options = createCompilerOptions();
String code = "function foo() {} var x = 3; foo(x);";
testSame(options, code);
options.inlineVariables = true;
test(options, code, "(function foo() {})(3);");
options.propertyRenaming = PropertyRenamingPolicy.HEURISTIC;
test(options, code, DefaultPassConfig.CANNOT_USE_PROTOTYPE_AND_VAR);
}
public void testInlineConstants() {
CompilerOptions options = createCompilerOptions();
String code = "function foo() {} var x = 3; foo(x); var YYY = 4; foo(YYY);";
testSame(options, code);
options.inlineConstantVars = true;
test(options, code, "function foo() {} var x = 3; foo(x); foo(4);");
}
public void testMinimizeExits() {
CompilerOptions options = createCompilerOptions();
String code =
"function f() {" +
" if (window.foo) return; window.h(); " +
"}";
testSame(options, code);
options.foldConstants = true;
test(
options, code,
"function f() {" +
" window.foo || window.h(); " +
"}");
}
public void testFoldConstants() {
CompilerOptions options = createCompilerOptions();
String code = "if (true) { window.foo(); }";
testSame(options, code);
options.foldConstants = true;
test(options, code, "window.foo();");
}
public void testRemoveUnreachableCode() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { return; f(); }";
testSame(options, code);
options.removeDeadCode = true;
test(options, code, "function f() {}");
}
public void testRemoveUnusedPrototypeProperties1() {
CompilerOptions options = createCompilerOptions();
String code = "function Foo() {} " +
"Foo.prototype.bar = function() { return new Foo(); };";
testSame(options, code);
options.removeUnusedPrototypeProperties = true;
test(options, code, "function Foo() {}");
}
public void testRemoveUnusedPrototypeProperties2() {
CompilerOptions options = createCompilerOptions();
String code = "function Foo() {} " +
"Foo.prototype.bar = function() { return new Foo(); };" +
"function f(x) { x.bar(); }";
testSame(options, code);
options.removeUnusedPrototypeProperties = true;
testSame(options, code);
options.removeUnusedVars = true;
test(options, code, "");
}
public void testSmartNamePass() {
CompilerOptions options = createCompilerOptions();
String code = "function Foo() { this.bar(); } " +
"Foo.prototype.bar = function() { return Foo(); };";
testSame(options, code);
options.smartNameRemoval = true;
test(options, code, "");
}
public void testDeadAssignmentsElimination() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { var x = 3; 4; x = 5; return x; } f(); ";
testSame(options, code);
options.deadAssignmentElimination = true;
testSame(options, code);
options.removeUnusedVars = true;
test(options, code, "function f() { var x = 3; 4; x = 5; return x; } f();");
}
public void testInlineFunctions() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { return 3; } f(); ";
testSame(options, code);
options.inlineFunctions = true;
test(options, code, "3;");
}
public void testRemoveUnusedVars1() {
CompilerOptions options = createCompilerOptions();
String code = "function f(x) {} f();";
testSame(options, code);
options.removeUnusedVars = true;
test(options, code, "function f() {} f();");
}
public void testRemoveUnusedVars2() {
CompilerOptions options = createCompilerOptions();
String code = "(function f(x) {})();var g = function() {}; g();";
testSame(options, code);
options.removeUnusedVars = true;
test(options, code, "(function() {})();var g = function() {}; g();");
options.anonymousFunctionNaming = AnonymousFunctionNamingPolicy.UNMAPPED;
test(options, code, "(function f() {})();var g = function $g$() {}; g();");
}
public void testCrossModuleCodeMotion() {
CompilerOptions options = createCompilerOptions();
String[] code = new String[] {
"var x = 1;",
"x;",
};
testSame(options, code);
options.crossModuleCodeMotion = true;
test(options, code, new String[] {
"",
"var x = 1; x;",
});
}
public void testCrossModuleMethodMotion() {
CompilerOptions options = createCompilerOptions();
String[] code = new String[] {
"var Foo = function() {}; Foo.prototype.bar = function() {};" +
"var x = new Foo();",
"x.bar();",
};
testSame(options, code);
options.crossModuleMethodMotion = true;
test(options, code, new String[] {
CrossModuleMethodMotion.STUB_DECLARATIONS +
"var Foo = function() {};" +
"Foo.prototype.bar=JSCompiler_stubMethod(0); var x=new Foo;",
"Foo.prototype.bar=JSCompiler_unstubMethod(0,function(){}); x.bar()",
});
}
public void testFlowSensitiveInlineVariables1() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { var x = 3; x = 5; return x; }";
testSame(options, code);
options.flowSensitiveInlineVariables = true;
test(options, code, "function f() { var x = 3; return 5; }");
String unusedVar = "function f() { var x; x = 5; return x; } f()";
test(options, unusedVar, "function f() { var x; return 5; } f()");
options.removeUnusedVars = true;
test(options, unusedVar, "function f() { return 5; } f()");
}
public void testFlowSensitiveInlineVariables2() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.SIMPLE_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
test(options,
"function f () {\n" +
" var ab = 0;\n" +
" ab += '-';\n" +
" alert(ab);\n" +
"}",
"function f () {\n" +
" alert('0-');\n" +
"}");
}
public void testCollapseAnonymousFunctions() {
CompilerOptions options = createCompilerOptions();
String code = "var f = function() {};";
testSame(options, code);
options.collapseAnonymousFunctions = true;
test(options, code, "function f() {}");
}
public void testMoveFunctionDeclarations() {
CompilerOptions options = createCompilerOptions();
String code = "var x = f(); function f() { return 3; }";
testSame(options, code);
options.moveFunctionDeclarations = true;
test(options, code, "function f() { return 3; } var x = f();");
}
public void testNameAnonymousFunctions() {
CompilerOptions options = createCompilerOptions();
String code = "var f = function() {};";
testSame(options, code);
options.anonymousFunctionNaming = AnonymousFunctionNamingPolicy.MAPPED;
test(options, code, "var f = function $() {}");
assertNotNull(lastCompiler.getResult().namedAnonFunctionMap);
options.anonymousFunctionNaming = AnonymousFunctionNamingPolicy.UNMAPPED;
test(options, code, "var f = function $f$() {}");
assertNull(lastCompiler.getResult().namedAnonFunctionMap);
}
public void testExtractPrototypeMemberDeclarations() {
CompilerOptions options = createCompilerOptions();
String code = "var f = function() {};";
String expected = "var a; var b = function() {}; a = b.prototype;";
for (int i = 0; i < 10; i++) {
code += "f.prototype.a = " + i + ";";
expected += "a.a = " + i + ";";
}
testSame(options, code);
options.extractPrototypeMemberDeclarations = true;
options.variableRenaming = VariableRenamingPolicy.ALL;
test(options, code, expected);
options.propertyRenaming = PropertyRenamingPolicy.HEURISTIC;
options.variableRenaming = VariableRenamingPolicy.OFF;
testSame(options, code);
}
public void testDevirtualizationAndExtractPrototypeMemberDeclarations() {
CompilerOptions options = createCompilerOptions();
options.devirtualizePrototypeMethods = true;
options.collapseAnonymousFunctions = true;
options.extractPrototypeMemberDeclarations = true;
options.variableRenaming = VariableRenamingPolicy.ALL;
String code = "var f = function() {};";
String expected = "var a; function b() {} a = b.prototype;";
for (int i = 0; i < 10; i++) {
code += "f.prototype.argz = function() {arguments};";
code += "f.prototype.devir" + i + " = function() {};";
char letter = (char) ('d' + i);
expected += "a.argz = function() {arguments};";
expected += "function " + letter + "(c){}";
}
code += "var F = new f(); F.argz();";
expected += "var n = new b(); n.argz();";
for (int i = 0; i < 10; i++) {
code += "F.devir" + i + "();";
char letter = (char) ('d' + i);
expected += letter + "(n);";
}
test(options, code, expected);
}
public void testCoalesceVariableNames() {
CompilerOptions options = createCompilerOptions();
String code = "function f() {var x = 3; var y = x; var z = y; return z;}";
testSame(options, code);
options.coalesceVariableNames = true;
test(options, code,
"function f() {var x = 3; x = x; x = x; return x;}");
}
public void testPropertyRenaming() {
CompilerOptions options = createCompilerOptions();
options.propertyAffinity = true;
String code =
"function f() { return this.foo + this['bar'] + this.Baz; }" +
"f.prototype.bar = 3; f.prototype.Baz = 3;";
String heuristic =
"function f() { return this.foo + this['bar'] + this.a; }" +
"f.prototype.bar = 3; f.prototype.a = 3;";
String aggHeuristic =
"function f() { return this.foo + this['b'] + this.a; } " +
"f.prototype.b = 3; f.prototype.a = 3;";
String all =
"function f() { return this.b + this['bar'] + this.a; }" +
"f.prototype.c = 3; f.prototype.a = 3;";
testSame(options, code);
options.propertyRenaming = PropertyRenamingPolicy.HEURISTIC;
test(options, code, heuristic);
options.propertyRenaming = PropertyRenamingPolicy.AGGRESSIVE_HEURISTIC;
test(options, code, aggHeuristic);
options.propertyRenaming = PropertyRenamingPolicy.ALL_UNQUOTED;
test(options, code, all);
}
public void testConvertToDottedProperties() {
CompilerOptions options = createCompilerOptions();
String code =
"function f() { return this['bar']; } f.prototype.bar = 3;";
String expected =
"function f() { return this.bar; } f.prototype.a = 3;";
testSame(options, code);
options.convertToDottedProperties = true;
options.propertyRenaming = PropertyRenamingPolicy.ALL_UNQUOTED;
test(options, code, expected);
}
public void testRewriteFunctionExpressions() {
CompilerOptions options = createCompilerOptions();
String code = "var a = function() {};";
String expected = "function JSCompiler_emptyFn(){return function(){}} " +
"var a = JSCompiler_emptyFn();";
for (int i = 0; i < 10; i++) {
code += "a = function() {};";
expected += "a = JSCompiler_emptyFn();";
}
testSame(options, code);
options.rewriteFunctionExpressions = true;
test(options, code, expected);
}
public void testAliasAllStrings() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { return 'a'; }";
String expected = "var $$S_a = 'a'; function f() { return $$S_a; }";
testSame(options, code);
options.aliasAllStrings = true;
test(options, code, expected);
}
public void testAliasExterns() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { return window + window + window + window; }";
String expected = "var GLOBAL_window = window;" +
"function f() { return GLOBAL_window + GLOBAL_window + " +
" GLOBAL_window + GLOBAL_window; }";
testSame(options, code);
options.aliasExternals = true;
test(options, code, expected);
}
public void testAliasKeywords() {
CompilerOptions options = createCompilerOptions();
String code =
"function f() { return true + true + true + true + true + true; }";
String expected = "var JSCompiler_alias_TRUE = true;" +
"function f() { return JSCompiler_alias_TRUE + " +
" JSCompiler_alias_TRUE + JSCompiler_alias_TRUE + " +
" JSCompiler_alias_TRUE + JSCompiler_alias_TRUE + " +
" JSCompiler_alias_TRUE; }";
testSame(options, code);
options.aliasKeywords = true;
test(options, code, expected);
}
public void testRenameVars1() {
CompilerOptions options = createCompilerOptions();
String code =
"var abc = 3; function f() { var xyz = 5; return abc + xyz; }";
String local = "var abc = 3; function f() { var a = 5; return abc + a; }";
String all = "var a = 3; function c() { var b = 5; return a + b; }";
testSame(options, code);
options.variableRenaming = VariableRenamingPolicy.LOCAL;
test(options, code, local);
options.variableRenaming = VariableRenamingPolicy.ALL;
test(options, code, all);
options.reserveRawExports = true;
}
public void testRenameVars2() {
CompilerOptions options = createCompilerOptions();
options.variableRenaming = VariableRenamingPolicy.ALL;
String code = "var abc = 3; function f() { window['a'] = 5; }";
String noexport = "var a = 3; function b() { window['a'] = 5; }";
String export = "var b = 3; function c() { window['a'] = 5; }";
options.reserveRawExports = false;
test(options, code, noexport);
options.reserveRawExports = true;
test(options, code, export);
}
public void testShadowVaribles() {
CompilerOptions options = createCompilerOptions();
options.variableRenaming = VariableRenamingPolicy.LOCAL;
options.shadowVariables = true;
String code = "var f = function(x) { return function(y) {}}";
String expected = "var f = function(a) { return function(a) {}}";
test(options, code, expected);
}
public void testRenameLabels() {
CompilerOptions options = createCompilerOptions();
String code = "longLabel: while (true) { break longLabel; }";
String expected = "a: while (true) { break a; }";
testSame(options, code);
options.labelRenaming = true;
test(options, code, expected);
}
public void testBadBreakStatementInIdeMode() {
// Ensure that type-checking doesn't crash, even if the CFG is malformed.
// This can happen in IDE mode.
CompilerOptions options = createCompilerOptions();
options.ideMode = true;
options.checkTypes = true;
test(options,
"function f() { try { } catch(e) { break; } }",
RhinoErrorReporter.PARSE_ERROR);
}
public void testIssue63SourceMap() {
CompilerOptions options = createCompilerOptions();
String code = "var a;";
options.skipAllPasses = true;
options.sourceMapOutputPath = "./src.map";
Compiler compiler = compile(options, code);
compiler.toSource();
}
public void testRegExp1() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = "/(a)/.test(\"a\");";
testSame(options, code);
options.computeFunctionSideEffects = true;
String expected = "";
test(options, code, expected);
}
public void testRegExp2() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = "/(a)/.test(\"a\");var a = RegExp.$1";
testSame(options, code);
options.computeFunctionSideEffects = true;
test(options, code, CheckRegExp.REGEXP_REFERENCE);
options.setWarningLevel(DiagnosticGroups.CHECK_REGEXP, CheckLevel.OFF);
testSame(options, code);
}
public void testFoldLocals1() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
// An external object, whose constructor has no side-effects,
// and whose method "go" only modifies the object.
String code = "new Widget().go();";
testSame(options, code);
options.computeFunctionSideEffects = true;
test(options, code, "");
}
public void testFoldLocals2() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
options.checkTypes = true;
// An external function that returns a local object that the
// method "go" that only modifies the object.
String code = "widgetToken().go();";
testSame(options, code);
options.computeFunctionSideEffects = true;
test(options, code, "widgetToken()");
}
public void testFoldLocals3() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
// A function "f" who returns a known local object, and a method that
// modifies only modifies that.
String definition = "function f(){return new Widget()}";
String call = "f().go();";
String code = definition + call;
testSame(options, code);
options.computeFunctionSideEffects = true;
// BROKEN
//test(options, code, definition);
testSame(options, code);
}
public void testFoldLocals4() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = "/** @constructor */\n"
+ "function InternalWidget(){this.x = 1;}"
+ "InternalWidget.prototype.internalGo = function (){this.x = 2};"
+ "new InternalWidget().internalGo();";
testSame(options, code);
options.computeFunctionSideEffects = true;
String optimized = ""
+ "function InternalWidget(){this.x = 1;}"
+ "InternalWidget.prototype.internalGo = function (){this.x = 2};";
test(options, code, optimized);
}
public void testFoldLocals5() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = ""
+ "function fn(){var a={};a.x={};return a}"
+ "fn().x.y = 1;";
// "fn" returns a unescaped local object, we should be able to fold it,
// but we don't currently.
String result = ""
+ "function fn(){var a={x:{}};return a}"
+ "fn().x.y = 1;";
test(options, code, result);
options.computeFunctionSideEffects = true;
test(options, code, result);
}
public void testFoldLocals6() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = ""
+ "function fn(){return {}}"
+ "fn().x.y = 1;";
testSame(options, code);
options.computeFunctionSideEffects = true;
testSame(options, code);
}
public void testFoldLocals7() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = ""
+ "function InternalWidget(){return [];}"
+ "Array.prototype.internalGo = function (){this.x = 2};"
+ "InternalWidget().internalGo();";
testSame(options, code);
options.computeFunctionSideEffects = true;
String optimized = ""
+ "function InternalWidget(){return [];}"
+ "Array.prototype.internalGo = function (){this.x = 2};";
test(options, code, optimized);
}
public void testVarDeclarationsIntoFor() {
CompilerOptions options = createCompilerOptions();
options.collapseVariableDeclarations = false;
String code = "var a = 1; for (var b = 2; ;) {}";
testSame(options, code);
options.collapseVariableDeclarations = false;
test(options, code, "for (var a = 1, b = 2; ;) {}");
}
public void testExploitAssigns() {
CompilerOptions options = createCompilerOptions();
options.collapseVariableDeclarations = false;
String code = "a = 1; b = a; c = b";
testSame(options, code);
options.collapseVariableDeclarations = true;
test(options, code, "c=b=a=1");
}
public void testRecoverOnBadExterns() throws Exception {
// This test is for a bug in a very narrow set of circumstances:
// 1) externs validation has to be off.
// 2) aliasExternals has to be on.
// 3) The user has to reference a "normal" variable in externs.
// This case is handled at checking time by injecting a
// synthetic extern variable, and adding a "@suppress {duplicate}" to
// the normal code at compile time. But optimizations may remove that
// annotation, so we need to make sure that the variable declarations
// are de-duped before that happens.
CompilerOptions options = createCompilerOptions();
options.aliasExternals = true;
externs = new JSSourceFile[] {
JSSourceFile.fromCode("externs", "extern.foo")
};
test(options,
"var extern; " +
"function f() { return extern + extern + extern + extern; }",
"var extern; " +
"function f() { return extern + extern + extern + extern; }",
VarCheck.UNDEFINED_EXTERN_VAR_ERROR);
}
public void testDuplicateVariablesInExterns() {
CompilerOptions options = createCompilerOptions();
options.checkSymbols = true;
externs = new JSSourceFile[] {
JSSourceFile.fromCode("externs",
"var externs = {}; /** @suppress {duplicate} */ var externs = {};")
};
testSame(options, "");
}
public void testLanguageMode() {
CompilerOptions options = createCompilerOptions();
options.setLanguageIn(LanguageMode.ECMASCRIPT3);
String code = "var a = {get f(){}}";
Compiler compiler = compile(options, code);
checkUnexpectedErrorsOrWarnings(compiler, 1);
assertEquals(
"JSC_PARSE_ERROR. Parse error. " +
"getters are not supported in Internet Explorer " +
"at i0 line 1 : 0",
compiler.getErrors()[0].toString());
options.setLanguageIn(LanguageMode.ECMASCRIPT5);
testSame(options, code);
options.setLanguageIn(LanguageMode.ECMASCRIPT5_STRICT);
testSame(options, code);
}
public void testLanguageMode2() {
CompilerOptions options = createCompilerOptions();
options.setLanguageIn(LanguageMode.ECMASCRIPT3);
options.setWarningLevel(DiagnosticGroups.ES5_STRICT, CheckLevel.OFF);
String code = "var a = 2; delete a;";
testSame(options, code);
options.setLanguageIn(LanguageMode.ECMASCRIPT5);
testSame(options, code);
options.setLanguageIn(LanguageMode.ECMASCRIPT5_STRICT);
test(options,
code,
code,
StrictModeCheck.DELETE_VARIABLE);
}
public void testIssue598() {
CompilerOptions options = createCompilerOptions();
options.setLanguageIn(LanguageMode.ECMASCRIPT5_STRICT);
WarningLevel.VERBOSE.setOptionsForWarningLevel(options);
options.setLanguageIn(LanguageMode.ECMASCRIPT5);
String code =
"'use strict';\n" +
"function App() {}\n" +
"App.prototype = {\n" +
" get appData() { return this.appData_; },\n" +
" set appData(data) { this.appData_ = data; }\n" +
"};";
Compiler compiler = compile(options, code);
testSame(options, code);
}
public void testCoaleseVariables() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = false;
options.coalesceVariableNames = true;
String code =
"function f(a) {" +
" if (a) {" +
" return a;" +
" } else {" +
" var b = a;" +
" return b;" +
" }" +
" return a;" +
"}";
String expected =
"function f(a) {" +
" if (a) {" +
" return a;" +
" } else {" +
" a = a;" +
" return a;" +
" }" +
" return a;" +
"}";
test(options, code, expected);
options.foldConstants = true;
options.coalesceVariableNames = false;
code =
"function f(a) {" +
" if (a) {" +
" return a;" +
" } else {" +
" var b = a;" +
" return b;" +
" }" +
" return a;" +
"}";
expected =
"function f(a) {" +
" if (!a) {" +
" var b = a;" +
" return b;" +
" }" +
" return a;" +
"}";
test(options, code, expected);
options.foldConstants = true;
options.coalesceVariableNames = true;
expected =
"function f(a) {" +
" return a;" +
"}";
test(options, code, expected);
}
public void testLateStatementFusion() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
test(options,
"while(a){a();if(b){b();b()}}",
"for(;a;)a(),b&&(b(),b())");
}
public void testLateConstantReordering() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
test(options,
"if (x < 1 || x > 1 || 1 < x || 1 > x) { alert(x) }",
" (1 > x || 1 < x || 1 < x || 1 > x) && alert(x) ");
}
public void testsyntheticBlockOnDeadAssignments() {
CompilerOptions options = createCompilerOptions();
options.deadAssignmentElimination = true;
options.removeUnusedVars = true;
options.syntheticBlockStartMarker = "START";
options.syntheticBlockEndMarker = "END";
test(options, "var x; x = 1; START(); x = 1;END();x()",
"var x; x = 1;{START();{x = 1}END()}x()");
}
public void testBug4152835() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
options.syntheticBlockStartMarker = "START";
options.syntheticBlockEndMarker = "END";
test(options, "START();END()", "{START();{}END()}");
}
public void testBug5786871() {
CompilerOptions options = createCompilerOptions();
options.ideMode = true;
test(options, "function () {}", RhinoErrorReporter.PARSE_ERROR);
}
public void testIssue378() {
CompilerOptions options = createCompilerOptions();
options.inlineVariables = true;
options.flowSensitiveInlineVariables = true;
testSame(options, "function f(c) {var f = c; arguments[0] = this;" +
" f.apply(this, arguments); return this;}");
}
public void testIssue550() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.SIMPLE_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
options.foldConstants = true;
options.inlineVariables = true;
options.flowSensitiveInlineVariables = true;
test(options,
"function f(h) {\n" +
" var a = h;\n" +
" a = a + 'x';\n" +
" a = a + 'y';\n" +
" return a;\n" +
"}",
"function f(a) {return a + 'xy'}");
}
public void testIssue284() {
CompilerOptions options = createCompilerOptions();
options.smartNameRemoval = true;
test(options,
"var goog = {};" +
"goog.inherits = function(x, y) {};" +
"var ns = {};" +
"/** @constructor */" +
"ns.PageSelectionModel = function() {};" +
"/** @constructor */" +
"ns.PageSelectionModel.FooEvent = function() {};" +
"/** @constructor */" +
"ns.PageSelectionModel.SelectEvent = function() {};" +
"goog.inherits(ns.PageSelectionModel.ChangeEvent," +
" ns.PageSelectionModel.FooEvent);",
"");
}
public void testCodingConvention() {
Compiler compiler = new Compiler();
compiler.initOptions(new CompilerOptions());
assertEquals(
compiler.getCodingConvention().getClass().toString(),
ClosureCodingConvention.class.toString());
}
public void testJQueryStringSplitLoops() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
test(options,
"var x=['1','2','3','4','5','6','7']",
"var x='1,2,3,4,5,6,7'.split(',')");
options = createCompilerOptions();
options.foldConstants = true;
options.computeFunctionSideEffects = false;
options.removeUnusedVars = true;
// If we do splits too early, it would add a sideeffect to x.
test(options,
"var x=['1','2','3','4','5','6','7']",
"");
}
public void testAlwaysRunSafetyCheck() {
CompilerOptions options = createCompilerOptions();
options.checkSymbols = false;
options.customPasses = ArrayListMultimap.create();
options.customPasses.put(
CustomPassExecutionTime.BEFORE_OPTIMIZATIONS,
new CompilerPass() {
@Override public void process(Node externs, Node root) {
Node var = root.getLastChild().getFirstChild();
assertEquals(Token.VAR, var.getType());
var.detachFromParent();
}
});
try {
test(options,
"var x = 3; function f() { return x + z; }",
"function f() { return x + z; }");
fail("Expected runtime exception");
} catch (RuntimeException e) {
assertTrue(e.getMessage().indexOf("Unexpected variable x") != -1);
}
}
public void testSuppressEs5StrictWarning() {
CompilerOptions options = createCompilerOptions();
options.setWarningLevel(DiagnosticGroups.ES5_STRICT, CheckLevel.WARNING);
testSame(options,
"/** @suppress{es5Strict} */\n" +
"function f() { var arguments; }");
}
public void testCheckProvidesWarning() {
CompilerOptions options = createCompilerOptions();
options.setWarningLevel(DiagnosticGroups.CHECK_PROVIDES, CheckLevel.WARNING);
options.setCheckProvides(CheckLevel.WARNING);
test(options,
"/** @constructor */\n" +
"function f() { var arguments; }",
DiagnosticType.warning("JSC_MISSING_PROVIDE", "missing goog.provide(''{0}'')"));
}
public void testSuppressCheckProvidesWarning() {
CompilerOptions options = createCompilerOptions();
options.setWarningLevel(DiagnosticGroups.CHECK_PROVIDES, CheckLevel.WARNING);
options.setCheckProvides(CheckLevel.WARNING);
testSame(options,
"/** @constructor\n" +
" * @suppress{checkProvides} */\n" +
"function f() { var arguments; }");
}
public void testRenamePrefixNamespace() {
String code =
"var x = {}; x.FOO = 5; x.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.collapseProperties = true;
options.renamePrefixNamespace = "_";
test(options, code, "_.x$FOO = 5; _.x$bar = 3;");
}
public void testRenamePrefixNamespaceActivatesMoveFunctionDeclarations() {
CompilerOptions options = createCompilerOptions();
String code = "var x = f; function f() { return 3; }";
testSame(options, code);
assertFalse(options.moveFunctionDeclarations);
options.renamePrefixNamespace = "_";
test(options, code, "_.f = function() { return 3; }; _.x = _.f;");
}
public void testBrokenNameSpace() {
CompilerOptions options = createCompilerOptions();
String code = "var goog; goog.provide('i.am.on.a.Horse');" +
"i.am.on.a.Horse = function() {};" +
"i.am.on.a.Horse.prototype.x = function() {};" +
"i.am.on.a.Boat.prototype.y = function() {}";
options.closurePass = true;
options.collapseProperties = true;
options.smartNameRemoval = true;
test(options, code, "");
}
public void testNamelessParameter() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.ADVANCED_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
String code =
"var impl_0;" +
"$load($init());" +
"function $load(){" +
" window['f'] = impl_0;" +
"}" +
"function $init() {" +
" impl_0 = {};" +
"}";
String result =
"window.f = {};";
test(options, code, result);
}
public void testHiddenSideEffect() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.ADVANCED_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
options.setAliasExternals(true);
String code =
"window.offsetWidth;";
String result =
"window.offsetWidth;";
test(options, code, result);
}
public void testNegativeZero() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.ADVANCED_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
test(options,
"function bar(x) { return x; }\n" +
"function foo(x) { print(x / bar(0));\n" +
" print(x / bar(-0)); }\n" +
"foo(3);",
"print(3/0);print(3/-0);");
}
public void testSingletonGetter1() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.ADVANCED_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
options.setCodingConvention(new ClosureCodingConvention());
test(options,
"/** @const */\n" +
"var goog = goog || {};\n" +
"goog.addSingletonGetter = function(ctor) {\n" +
" ctor.getInstance = function() {\n" +
" return ctor.instance_ || (ctor.instance_ = new ctor());\n" +
" };\n" +
"};" +
"function Foo() {}\n" +
"goog.addSingletonGetter(Foo);" +
"Foo.prototype.bar = 1;" +
"function Bar() {}\n" +
"goog.addSingletonGetter(Bar);" +
"Bar.prototype.bar = 1;",
"");
}
public void testIncompleteFunction() {
CompilerOptions options = createCompilerOptions();
options.ideMode = true;
DiagnosticType[] warnings = new DiagnosticType[]{
RhinoErrorReporter.PARSE_ERROR,
RhinoErrorReporter.PARSE_ERROR,
RhinoErrorReporter.PARSE_ERROR,
RhinoErrorReporter.PARSE_ERROR};
test(options,
new String[] { "var foo = {bar: function(e) }" },
new String[] { "var foo = {bar: function(e){}};" },
warnings
);
}
private void testSame(CompilerOptions options, String original) {
testSame(options, new String[] { original });
}
private void testSame(CompilerOptions options, String[] original) {
test(options, original, original);
}
/**
* Asserts that when compiling with the given compiler options,
* {@code original} is transformed into {@code compiled}.
*/
private void test(CompilerOptions options,
String original, String compiled) {
test(options, new String[] { original }, new String[] { compiled });
}
/**
* Asserts that when compiling with the given compiler options,
* {@code original} is transformed into {@code compiled}.
*/
private void test(CompilerOptions options,
String[] original, String[] compiled) {
Compiler compiler = compile(options, original);
assertEquals("Expected no warnings or errors\n" +
"Errors: \n" + Joiner.on("\n").join(compiler.getErrors()) +
"Warnings: \n" + Joiner.on("\n").join(compiler.getWarnings()),
0, compiler.getErrors().length + compiler.getWarnings().length);
Node root = compiler.getRoot().getLastChild();
Node expectedRoot = parse(compiled, options);
String explanation = expectedRoot.checkTreeEquals(root);
assertNull("\nExpected: " + compiler.toSource(expectedRoot) +
"\nResult: " + compiler.toSource(root) +
"\n" + explanation, explanation);
}
/**
* Asserts that when compiling with the given compiler options,
* there is an error or warning.
*/
private void test(CompilerOptions options,
String original, DiagnosticType warning) {
test(options, new String[] { original }, warning);
}
private void test(CompilerOptions options,
String original, String compiled, DiagnosticType warning) {
test(options, new String[] { original }, new String[] { compiled },
warning);
}
private void test(CompilerOptions options,
String[] original, DiagnosticType warning) {
test(options, original, null, warning);
}
/**
* Asserts that when compiling with the given compiler options,
* there is an error or warning.
*/
private void test(CompilerOptions options,
String[] original, String[] compiled, DiagnosticType warning) {
Compiler compiler = compile(options, original);
checkUnexpectedErrorsOrWarnings(compiler, 1);
assertEquals("Expected exactly one warning or error",
1, compiler.getErrors().length + compiler.getWarnings().length);
if (compiler.getErrors().length > 0) {
assertEquals(warning, compiler.getErrors()[0].getType());
} else {
assertEquals(warning, compiler.getWarnings()[0].getType());
}
if (compiled != null) {
Node root = compiler.getRoot().getLastChild();
Node expectedRoot = parse(compiled, options);
String explanation = expectedRoot.checkTreeEquals(root);
assertNull("\nExpected: " + compiler.toSource(expectedRoot) +
"\nResult: " + compiler.toSource(root) +
"\n" + explanation, explanation);
}
}
/**
* Asserts that when compiling with the given compiler options,
* there is an error or warning.
*/
private void test(CompilerOptions options,
String[] original, String[] compiled, DiagnosticType[] warnings) {
Compiler compiler = compile(options, original);
checkUnexpectedErrorsOrWarnings(compiler, warnings.length);
if (compiled != null) {
Node root = compiler.getRoot().getLastChild();
Node expectedRoot = parse(compiled, options);
String explanation = expectedRoot.checkTreeEquals(root);
assertNull("\nExpected: " + compiler.toSource(expectedRoot) +
"\nResult: " + compiler.toSource(root) +
"\n" + explanation, explanation);
}
}
private void checkUnexpectedErrorsOrWarnings(
Compiler compiler, int expected) {
int actual = compiler.getErrors().length + compiler.getWarnings().length;
if (actual != expected) {
String msg = "";
for (JSError err : compiler.getErrors()) {
msg += "Error:" + err.toString() + "\n";
}
for (JSError err : compiler.getWarnings()) {
msg += "Warning:" + err.toString() + "\n";
}
assertEquals("Unexpected warnings or errors.\n "+ msg,
expected, actual);
}
}
private Compiler compile(CompilerOptions options, String original) {
return compile(options, new String[] { original });
}
private Compiler compile(CompilerOptions options, String[] original) {
Compiler compiler = lastCompiler = new Compiler();
JSSourceFile[] inputs = new JSSourceFile[original.length];
for (int i = 0; i < original.length; i++) {
inputs[i] = JSSourceFile.fromCode("input" + i, original[i]);
}
compiler.compile(
externs, CompilerTestCase.createModuleChain(original), options);
return compiler;
}
private Node parse(String[] original, CompilerOptions options) {
Compiler compiler = new Compiler();
JSSourceFile[] inputs = new JSSourceFile[original.length];
for (int i = 0; i < inputs.length; i++) {
inputs[i] = JSSourceFile.fromCode("input" + i, original[i]);
}
compiler.init(externs, inputs, options);
checkUnexpectedErrorsOrWarnings(compiler, 0);
Node all = compiler.parseInputs();
checkUnexpectedErrorsOrWarnings(compiler, 0);
Node n = all.getLastChild();
Node externs = all.getFirstChild();
(new CreateSyntheticBlocks(
compiler, "synStart", "synEnd")).process(externs, n);
(new Normalize(compiler, false)).process(externs, n);
(MakeDeclaredNamesUnique.getContextualRenameInverter(compiler)).process(
externs, n);
(new Denormalize(compiler)).process(externs, n);
return n;
}
/** Creates a CompilerOptions object with google coding conventions. */
private CompilerOptions createCompilerOptions() {
CompilerOptions options = new CompilerOptions();
options.setCodingConvention(new GoogleCodingConvention());
return options;
}
} |
||
public void testIssue700() throws Exception {
testTypes(
"/**\n" +
" * @param {{text: string}} opt_data\n" +
" * @return {string}\n" +
" */\n" +
"function temp1(opt_data) {\n" +
" return opt_data.text;\n" +
"}\n" +
"\n" +
"/**\n" +
" * @param {{activity: (boolean|number|string|null|Object)}} opt_data\n" +
" * @return {string}\n" +
" */\n" +
"function temp2(opt_data) {\n" +
" /** @notypecheck */\n" +
" function __inner() {\n" +
" return temp1(opt_data.activity);\n" +
" }\n" +
" return __inner();\n" +
"}\n" +
"\n" +
"/**\n" +
" * @param {{n: number, text: string, b: boolean}} opt_data\n" +
" * @return {string}\n" +
" */\n" +
"function temp3(opt_data) {\n" +
" return 'n: ' + opt_data.n + ', t: ' + opt_data.text + '.';\n" +
"}\n" +
"\n" +
"function callee() {\n" +
" var output = temp3({\n" +
" n: 0,\n" +
" text: 'a string',\n" +
" b: true\n" +
" })\n" +
" alert(output);\n" +
"}\n" +
"\n" +
"callee();");
} | com.google.javascript.jscomp.TypeCheckTest::testIssue700 | test/com/google/javascript/jscomp/TypeCheckTest.java | 5,815 | test/com/google/javascript/jscomp/TypeCheckTest.java | testIssue700 | /*
* Copyright 2006 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import com.google.common.base.Joiner;
import com.google.common.collect.Lists;
import com.google.javascript.jscomp.Scope.Var;
import com.google.javascript.rhino.InputId;
import com.google.javascript.rhino.Node;
import com.google.javascript.rhino.Token;
import com.google.javascript.rhino.jstype.FunctionType;
import com.google.javascript.rhino.jstype.JSType;
import com.google.javascript.rhino.jstype.JSTypeNative;
import com.google.javascript.rhino.jstype.ObjectType;
import java.util.Arrays;
import java.util.List;
/**
* Tests {@link TypeCheck}.
*
*/
public class TypeCheckTest extends CompilerTypeTestCase {
private CheckLevel reportMissingOverrides = CheckLevel.WARNING;
@Override
public void setUp() throws Exception {
super.setUp();
reportMissingOverrides = CheckLevel.WARNING;
}
public void testInitialTypingScope() {
Scope s = new TypedScopeCreator(compiler,
CodingConventions.getDefault()).createInitialScope(
new Node(Token.BLOCK));
assertEquals(ARRAY_FUNCTION_TYPE, s.getVar("Array").getType());
assertEquals(BOOLEAN_OBJECT_FUNCTION_TYPE,
s.getVar("Boolean").getType());
assertEquals(DATE_FUNCTION_TYPE, s.getVar("Date").getType());
assertEquals(ERROR_FUNCTION_TYPE, s.getVar("Error").getType());
assertEquals(EVAL_ERROR_FUNCTION_TYPE,
s.getVar("EvalError").getType());
assertEquals(NUMBER_OBJECT_FUNCTION_TYPE,
s.getVar("Number").getType());
assertEquals(OBJECT_FUNCTION_TYPE, s.getVar("Object").getType());
assertEquals(RANGE_ERROR_FUNCTION_TYPE,
s.getVar("RangeError").getType());
assertEquals(REFERENCE_ERROR_FUNCTION_TYPE,
s.getVar("ReferenceError").getType());
assertEquals(REGEXP_FUNCTION_TYPE, s.getVar("RegExp").getType());
assertEquals(STRING_OBJECT_FUNCTION_TYPE,
s.getVar("String").getType());
assertEquals(SYNTAX_ERROR_FUNCTION_TYPE,
s.getVar("SyntaxError").getType());
assertEquals(TYPE_ERROR_FUNCTION_TYPE,
s.getVar("TypeError").getType());
assertEquals(URI_ERROR_FUNCTION_TYPE,
s.getVar("URIError").getType());
}
public void testTypeCheck1() throws Exception {
testTypes("/**@return {void}*/function foo(){ if (foo()) return; }");
}
public void testTypeCheck2() throws Exception {
testTypes("/**@return {void}*/function foo(){ var x=foo(); x--; }",
"increment/decrement\n" +
"found : undefined\n" +
"required: number");
}
public void testTypeCheck4() throws Exception {
testTypes("/**@return {void}*/function foo(){ !foo(); }");
}
public void testTypeCheck5() throws Exception {
testTypes("/**@return {void}*/function foo(){ var a = +foo(); }",
"sign operator\n" +
"found : undefined\n" +
"required: number");
}
public void testTypeCheck6() throws Exception {
testTypes(
"/**@return {void}*/function foo(){" +
"/** @type {undefined|number} */var a;if (a == foo())return;}");
}
public void testTypeCheck8() throws Exception {
testTypes("/**@return {void}*/function foo(){do {} while (foo());}");
}
public void testTypeCheck9() throws Exception {
testTypes("/**@return {void}*/function foo(){while (foo());}");
}
public void testTypeCheck10() throws Exception {
testTypes("/**@return {void}*/function foo(){for (;foo(););}");
}
public void testTypeCheck11() throws Exception {
testTypes("/**@type !Number */var a;" +
"/**@type !String */var b;" +
"a = b;",
"assignment\n" +
"found : String\n" +
"required: Number");
}
public void testTypeCheck12() throws Exception {
testTypes("/**@return {!Object}*/function foo(){var a = 3^foo();}",
"bad right operand to bitwise operator\n" +
"found : Object\n" +
"required: (boolean|null|number|string|undefined)");
}
public void testTypeCheck13() throws Exception {
testTypes("/**@type {!Number|!String}*/var i; i=/xx/;",
"assignment\n" +
"found : RegExp\n" +
"required: (Number|String)");
}
public void testTypeCheck14() throws Exception {
testTypes("/**@param opt_a*/function foo(opt_a){}");
}
public void testTypeCheck15() throws Exception {
testTypes("/**@type {Number|null} */var x;x=null;x=10;",
"assignment\n" +
"found : number\n" +
"required: (Number|null)");
}
public void testTypeCheck16() throws Exception {
testTypes("/**@type {Number|null} */var x='';",
"initializing variable\n" +
"found : string\n" +
"required: (Number|null)");
}
public void testTypeCheck17() throws Exception {
testTypes("/**@return {Number}\n@param {Number} opt_foo */\n" +
"function a(opt_foo){\nreturn /**@type {Number}*/(opt_foo);\n}");
}
public void testTypeCheck18() throws Exception {
testTypes("/**@return {RegExp}\n*/\n function a(){return new RegExp();}");
}
public void testTypeCheck19() throws Exception {
testTypes("/**@return {Array}\n*/\n function a(){return new Array();}");
}
public void testTypeCheck20() throws Exception {
testTypes("/**@return {Date}\n*/\n function a(){return new Date();}");
}
public void testTypeCheckBasicDowncast() throws Exception {
testTypes("/** @constructor */function foo() {}\n" +
"/** @type {Object} */ var bar = new foo();\n");
}
public void testTypeCheckNoDowncastToNumber() throws Exception {
testTypes("/** @constructor */function foo() {}\n" +
"/** @type {!Number} */ var bar = new foo();\n",
"initializing variable\n" +
"found : foo\n" +
"required: Number");
}
public void testTypeCheck21() throws Exception {
testTypes("/** @type Array.<String> */var foo;");
}
public void testTypeCheck22() throws Exception {
testTypes("/** @param {Element|Object} p */\nfunction foo(p){}\n" +
"/** @constructor */function Element(){}\n" +
"/** @type {Element|Object} */var v;\n" +
"foo(v);\n");
}
public void testTypeCheck23() throws Exception {
testTypes("/** @type {(Object,Null)} */var foo; foo = null;");
}
public void testTypeCheck24() throws Exception {
testTypes("/** @constructor */function MyType(){}\n" +
"/** @type {(MyType,Null)} */var foo; foo = null;");
}
public void testTypeCheckDefaultExterns() throws Exception {
testTypes("/** @param {string} x */ function f(x) {}" +
"f([].length);" ,
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testTypeCheckCustomExterns() throws Exception {
testTypes(
DEFAULT_EXTERNS + "/** @type {boolean} */ Array.prototype.oogabooga;",
"/** @param {string} x */ function f(x) {}" +
"f([].oogabooga);" ,
"actual parameter 1 of f does not match formal parameter\n" +
"found : boolean\n" +
"required: string", false);
}
public void testTypeCheckCustomExterns2() throws Exception {
testTypes(
DEFAULT_EXTERNS + "/** @enum {string} */ var Enum = {FOO: 1, BAR: 1};",
"/** @param {Enum} x */ function f(x) {} f(Enum.FOO); f(true);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : boolean\n" +
"required: Enum.<string>",
false);
}
public void testParameterizedArray1() throws Exception {
testTypes("/** @param {!Array.<number>} a\n" +
"* @return {string}\n" +
"*/ var f = function(a) { return a[0]; };",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testParameterizedArray2() throws Exception {
testTypes("/** @param {!Array.<!Array.<number>>} a\n" +
"* @return {number}\n" +
"*/ var f = function(a) { return a[0]; };",
"inconsistent return type\n" +
"found : Array.<number>\n" +
"required: number");
}
public void testParameterizedArray3() throws Exception {
testTypes("/** @param {!Array.<number>} a\n" +
"* @return {number}\n" +
"*/ var f = function(a) { a[1] = 0; return a[0]; };");
}
public void testParameterizedArray4() throws Exception {
testTypes("/** @param {!Array.<number>} a\n" +
"*/ var f = function(a) { a[0] = 'a'; };",
"assignment\n" +
"found : string\n" +
"required: number");
}
public void testParameterizedArray5() throws Exception {
testTypes("/** @param {!Array.<*>} a\n" +
"*/ var f = function(a) { a[0] = 'a'; };");
}
public void testParameterizedArray6() throws Exception {
testTypes("/** @param {!Array.<*>} a\n" +
"* @return {string}\n" +
"*/ var f = function(a) { return a[0]; };",
"inconsistent return type\n" +
"found : *\n" +
"required: string");
}
public void testParameterizedArray7() throws Exception {
testTypes("/** @param {?Array.<number>} a\n" +
"* @return {string}\n" +
"*/ var f = function(a) { return a[0]; };",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testParameterizedObject1() throws Exception {
testTypes("/** @param {!Object.<number>} a\n" +
"* @return {string}\n" +
"*/ var f = function(a) { return a[0]; };",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testParameterizedObject2() throws Exception {
testTypes("/** @param {!Object.<string,number>} a\n" +
"* @return {string}\n" +
"*/ var f = function(a) { return a['x']; };",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testParameterizedObject3() throws Exception {
testTypes("/** @param {!Object.<number,string>} a\n" +
"* @return {string}\n" +
"*/ var f = function(a) { return a['x']; };",
"restricted index type\n" +
"found : string\n" +
"required: number");
}
public void testParameterizedObject4() throws Exception {
testTypes("/** @enum {string} */ var E = {A: 'a', B: 'b'};\n" +
"/** @param {!Object.<E,string>} a\n" +
"* @return {string}\n" +
"*/ var f = function(a) { return a['x']; };",
"restricted index type\n" +
"found : string\n" +
"required: E.<string>");
}
public void testParameterizedObject5() throws Exception {
testTypes("/** @constructor */ function F() {" +
" /** @type {Object.<number, string>} */ this.numbers = {};" +
"}" +
"(new F()).numbers['ten'] = '10';",
"restricted index type\n" +
"found : string\n" +
"required: number");
}
public void testUnionOfFunctionAndType() throws Exception {
testTypes("/** @type {null|(function(Number):void)} */ var a;" +
"/** @type {(function(Number):void)|null} */ var b = null; a = b;");
}
public void testOptionalParameterComparedToUndefined() throws Exception {
testTypes("/**@param opt_a {Number}*/function foo(opt_a)" +
"{if (opt_a==undefined) var b = 3;}");
}
public void testOptionalAllType() throws Exception {
testTypes("/** @param {*} opt_x */function f(opt_x) { return opt_x }\n" +
"/** @type {*} */var y;\n" +
"f(y);");
}
public void testOptionalUnknownNamedType() throws Exception {
testTypes("/** @param {!T} opt_x\n@return {undefined} */\n" +
"function f(opt_x) { return opt_x; }\n" +
"/** @constructor */var T = function() {};",
"inconsistent return type\n" +
"found : (T|undefined)\n" +
"required: undefined");
}
public void testOptionalArgFunctionParam() throws Exception {
testTypes("/** @param {function(number=)} a */" +
"function f(a) {a()};");
}
public void testOptionalArgFunctionParam2() throws Exception {
testTypes("/** @param {function(number=)} a */" +
"function f(a) {a(3)};");
}
public void testOptionalArgFunctionParam3() throws Exception {
testTypes("/** @param {function(number=)} a */" +
"function f(a) {a(undefined)};");
}
public void testOptionalArgFunctionParam4() throws Exception {
String expectedWarning = "Function a: called with 2 argument(s). " +
"Function requires at least 0 argument(s) and no more than 1 " +
"argument(s).";
testTypes("/** @param {function(number=)} a */function f(a) {a(3,4)};",
expectedWarning, false);
}
public void testOptionalArgFunctionParamError() throws Exception {
String expectedWarning =
"Bad type annotation. variable length argument must be last";
testTypes("/** @param {function(...[number], number=)} a */" +
"function f(a) {};", expectedWarning, false);
}
public void testOptionalNullableArgFunctionParam() throws Exception {
testTypes("/** @param {function(?number=)} a */" +
"function f(a) {a()};");
}
public void testOptionalNullableArgFunctionParam2() throws Exception {
testTypes("/** @param {function(?number=)} a */" +
"function f(a) {a(null)};");
}
public void testOptionalNullableArgFunctionParam3() throws Exception {
testTypes("/** @param {function(?number=)} a */" +
"function f(a) {a(3)};");
}
public void testOptionalArgFunctionReturn() throws Exception {
testTypes("/** @return {function(number=)} */" +
"function f() { return function(opt_x) { }; };" +
"f()()");
}
public void testOptionalArgFunctionReturn2() throws Exception {
testTypes("/** @return {function(Object=)} */" +
"function f() { return function(opt_x) { }; };" +
"f()({})");
}
public void testBooleanType() throws Exception {
testTypes("/**@type {boolean} */var x = 1 < 2;");
}
public void testBooleanReduction1() throws Exception {
testTypes("/**@type {string} */var x; x = null || \"a\";");
}
public void testBooleanReduction2() throws Exception {
// It's important for the type system to recognize that in no case
// can the boolean expression evaluate to a boolean value.
testTypes("/** @param {string} s\n @return {string} */" +
"(function(s) { return ((s == 'a') && s) || 'b'; })");
}
public void testBooleanReduction3() throws Exception {
testTypes("/** @param {string} s\n @return {string?} */" +
"(function(s) { return s && null && 3; })");
}
public void testBooleanReduction4() throws Exception {
testTypes("/** @param {Object} x\n @return {Object} */" +
"(function(x) { return null || x || null ; })");
}
public void testBooleanReduction5() throws Exception {
testTypes("/**\n" +
"* @param {Array|string} x\n" +
"* @return {string?}\n" +
"*/\n" +
"var f = function(x) {\n" +
"if (!x || typeof x == 'string') {\n" +
"return x;\n" +
"}\n" +
"return null;\n" +
"};");
}
public void testBooleanReduction6() throws Exception {
testTypes("/**\n" +
"* @param {Array|string|null} x\n" +
"* @return {string?}\n" +
"*/\n" +
"var f = function(x) {\n" +
"if (!(x && typeof x != 'string')) {\n" +
"return x;\n" +
"}\n" +
"return null;\n" +
"};");
}
public void testBooleanReduction7() throws Exception {
testTypes("/** @constructor */var T = function() {};\n" +
"/**\n" +
"* @param {Array|T} x\n" +
"* @return {null}\n" +
"*/\n" +
"var f = function(x) {\n" +
"if (!x) {\n" +
"return x;\n" +
"}\n" +
"return null;\n" +
"};");
}
public void testNullAnd() throws Exception {
testTypes("/** @type null */var x;\n" +
"/** @type number */var r = x && x;",
"initializing variable\n" +
"found : null\n" +
"required: number");
}
public void testNullOr() throws Exception {
testTypes("/** @type null */var x;\n" +
"/** @type number */var r = x || x;",
"initializing variable\n" +
"found : null\n" +
"required: number");
}
public void testBooleanPreservation1() throws Exception {
testTypes("/**@type {string} */var x = \"a\";" +
"x = ((x == \"a\") && x) || x == \"b\";",
"assignment\n" +
"found : (boolean|string)\n" +
"required: string");
}
public void testBooleanPreservation2() throws Exception {
testTypes("/**@type {string} */var x = \"a\"; x = (x == \"a\") || x;",
"assignment\n" +
"found : (boolean|string)\n" +
"required: string");
}
public void testBooleanPreservation3() throws Exception {
testTypes("/** @param {Function?} x\n @return {boolean?} */" +
"function f(x) { return x && x == \"a\"; }",
"condition always evaluates to false\n" +
"left : Function\n" +
"right: string");
}
public void testBooleanPreservation4() throws Exception {
testTypes("/** @param {Function?|boolean} x\n @return {boolean} */" +
"function f(x) { return x && x == \"a\"; }",
"inconsistent return type\n" +
"found : (boolean|null)\n" +
"required: boolean");
}
public void testTypeOfReduction1() throws Exception {
testTypes("/** @param {string|number} x\n @return {string} */ " +
"function f(x) { return typeof x == 'number' ? String(x) : x; }");
}
public void testTypeOfReduction2() throws Exception {
testTypes("/** @param {string|number} x\n @return {string} */ " +
"function f(x) { return typeof x != 'string' ? String(x) : x; }");
}
public void testTypeOfReduction3() throws Exception {
testTypes("/** @param {number|null} x\n @return {number} */ " +
"function f(x) { return typeof x == 'object' ? 1 : x; }");
}
public void testTypeOfReduction4() throws Exception {
testTypes("/** @param {Object|undefined} x\n @return {Object} */ " +
"function f(x) { return typeof x == 'undefined' ? {} : x; }");
}
public void testTypeOfReduction5() throws Exception {
testTypes("/** @enum {string} */ var E = {A: 'a', B: 'b'};\n" +
"/** @param {!E|number} x\n @return {string} */ " +
"function f(x) { return typeof x != 'number' ? x : 'a'; }");
}
public void testTypeOfReduction6() throws Exception {
testTypes("/** @param {number|string} x\n@return {string} */\n" +
"function f(x) {\n" +
"return typeof x == 'string' && x.length == 3 ? x : 'a';\n" +
"}");
}
public void testTypeOfReduction7() throws Exception {
testTypes("/** @return {string} */var f = function(x) { " +
"return typeof x == 'number' ? x : 'a'; }",
"inconsistent return type\n" +
"found : (number|string)\n" +
"required: string");
}
public void testTypeOfReduction8() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @param {number|string} x\n@return {string} */\n" +
"function f(x) {\n" +
"return goog.isString(x) && x.length == 3 ? x : 'a';\n" +
"}", null);
}
public void testTypeOfReduction9() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @param {!Array|string} x\n@return {string} */\n" +
"function f(x) {\n" +
"return goog.isArray(x) ? 'a' : x;\n" +
"}", null);
}
public void testTypeOfReduction10() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @param {Array|string} x\n@return {Array} */\n" +
"function f(x) {\n" +
"return goog.isArray(x) ? x : [];\n" +
"}", null);
}
public void testTypeOfReduction11() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @param {Array|string} x\n@return {Array} */\n" +
"function f(x) {\n" +
"return goog.isObject(x) ? x : [];\n" +
"}", null);
}
public void testTypeOfReduction12() throws Exception {
testTypes("/** @enum {string} */ var E = {A: 'a', B: 'b'};\n" +
"/** @param {E|Array} x\n @return {Array} */ " +
"function f(x) { return typeof x == 'object' ? x : []; }");
}
public void testTypeOfReduction13() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @enum {string} */ var E = {A: 'a', B: 'b'};\n" +
"/** @param {E|Array} x\n@return {Array} */ " +
"function f(x) { return goog.isObject(x) ? x : []; }", null);
}
public void testTypeOfReduction14() throws Exception {
// Don't do type inference on GETELEMs.
testClosureTypes(
CLOSURE_DEFS +
"function f(x) { " +
" return goog.isString(arguments[0]) ? arguments[0] : 0;" +
"}", null);
}
public void testTypeOfReduction15() throws Exception {
// Don't do type inference on GETELEMs.
testClosureTypes(
CLOSURE_DEFS +
"function f(x) { " +
" return typeof arguments[0] == 'string' ? arguments[0] : 0;" +
"}", null);
}
public void testQualifiedNameReduction1() throws Exception {
testTypes("var x = {}; /** @type {string?} */ x.a = 'a';\n" +
"/** @return {string} */ var f = function() {\n" +
"return x.a ? x.a : 'a'; }");
}
public void testQualifiedNameReduction2() throws Exception {
testTypes("/** @param {string?} a\n@constructor */ var T = " +
"function(a) {this.a = a};\n" +
"/** @return {string} */ T.prototype.f = function() {\n" +
"return this.a ? this.a : 'a'; }");
}
public void testQualifiedNameReduction3() throws Exception {
testTypes("/** @param {string|Array} a\n@constructor */ var T = " +
"function(a) {this.a = a};\n" +
"/** @return {string} */ T.prototype.f = function() {\n" +
"return typeof this.a == 'string' ? this.a : 'a'; }");
}
public void testQualifiedNameReduction4() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @param {string|Array} a\n@constructor */ var T = " +
"function(a) {this.a = a};\n" +
"/** @return {string} */ T.prototype.f = function() {\n" +
"return goog.isString(this.a) ? this.a : 'a'; }", null);
}
public void testQualifiedNameReduction5a() throws Exception {
testTypes("var x = {/** @type {string} */ a:'b' };\n" +
"/** @return {string} */ var f = function() {\n" +
"return x.a; }");
}
public void testQualifiedNameReduction5b() throws Exception {
testTypes(
"var x = {/** @type {number} */ a:12 };\n" +
"/** @return {string} */\n" +
"var f = function() {\n" +
" return x.a;\n" +
"}",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testQualifiedNameReduction5c() throws Exception {
testTypes(
"/** @return {string} */ var f = function() {\n" +
"var x = {/** @type {number} */ a:0 };\n" +
"return (x.a) ? (x.a) : 'a'; }",
"inconsistent return type\n" +
"found : (number|string)\n" +
"required: string");
}
public void testQualifiedNameReduction6() throws Exception {
testTypes(
"/** @return {string} */ var f = function() {\n" +
"var x = {/** @return {string?} */ get a() {return 'a'}};\n" +
"return x.a ? x.a : 'a'; }");
}
public void testQualifiedNameReduction7() throws Exception {
testTypes(
"/** @return {string} */ var f = function() {\n" +
"var x = {/** @return {number} */ get a() {return 12}};\n" +
"return x.a; }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testQualifiedNameReduction7a() throws Exception {
// It would be nice to find a way to make this an error.
testTypes(
"/** @return {string} */ var f = function() {\n" +
"var x = {get a() {return 12}};\n" +
"return x.a; }");
}
public void testQualifiedNameReduction8() throws Exception {
testTypes(
"/** @return {string} */ var f = function() {\n" +
"var x = {get a() {return 'a'}};\n" +
"return x.a ? x.a : 'a'; }");
}
public void testQualifiedNameReduction9() throws Exception {
testTypes(
"/** @return {string} */ var f = function() {\n" +
"var x = { /** @param {string} b */ set a(b) {}};\n" +
"return x.a ? x.a : 'a'; }");
}
public void testQualifiedNameReduction10() throws Exception {
// TODO(johnlenz): separate setter property types from getter property
// types.
testTypes(
"/** @return {string} */ var f = function() {\n" +
"var x = { /** @param {number} b */ set a(b) {}};\n" +
"return x.a ? x.a : 'a'; }",
"inconsistent return type\n" +
"found : (number|string)\n" +
"required: string");
}
public void testObjLitDef1a() throws Exception {
testTypes(
"var x = {/** @type {number} */ a:12 };\n" +
"x.a = 'a';",
"assignment to property a of x\n" +
"found : string\n" +
"required: number");
}
public void testObjLitDef1b() throws Exception {
testTypes(
"function f(){" +
"var x = {/** @type {number} */ a:12 };\n" +
"x.a = 'a';" +
"};\n" +
"f();",
"assignment to property a of x\n" +
"found : string\n" +
"required: number");
}
public void testObjLitDef2a() throws Exception {
testTypes(
"var x = {/** @param {number} b */ set a(b){} };\n" +
"x.a = 'a';",
"assignment to property a of x\n" +
"found : string\n" +
"required: number");
}
public void testObjLitDef2b() throws Exception {
testTypes(
"function f(){" +
"var x = {/** @param {number} b */ set a(b){} };\n" +
"x.a = 'a';" +
"};\n" +
"f();",
"assignment to property a of x\n" +
"found : string\n" +
"required: number");
}
public void testObjLitDef3a() throws Exception {
testTypes(
"/** @type {string} */ var y;\n" +
"var x = {/** @return {number} */ get a(){} };\n" +
"y = x.a;",
"assignment\n" +
"found : number\n" +
"required: string");
}
public void testObjLitDef3b() throws Exception {
testTypes(
"/** @type {string} */ var y;\n" +
"function f(){" +
"var x = {/** @return {number} */ get a(){} };\n" +
"y = x.a;" +
"};\n" +
"f();",
"assignment\n" +
"found : number\n" +
"required: string");
}
public void testObjLitDef4() throws Exception {
testTypes(
"var x = {" +
"/** @return {number} */ a:12 };\n",
"assignment to property a of {a: function (): number}\n" +
"found : number\n" +
"required: function (): number");
}
public void testObjLitDef5() throws Exception {
testTypes(
"var x = {};\n" +
"/** @return {number} */ x.a = 12;\n",
"assignment to property a of x\n" +
"found : number\n" +
"required: function (): number");
}
public void testInstanceOfReduction1() throws Exception {
testTypes("/** @constructor */ var T = function() {};\n" +
"/** @param {T|string} x\n@return {T} */\n" +
"var f = function(x) {\n" +
"if (x instanceof T) { return x; } else { return new T(); }\n" +
"};");
}
public void testInstanceOfReduction2() throws Exception {
testTypes("/** @constructor */ var T = function() {};\n" +
"/** @param {!T|string} x\n@return {string} */\n" +
"var f = function(x) {\n" +
"if (x instanceof T) { return ''; } else { return x; }\n" +
"};");
}
public void testUndeclaredGlobalProperty1() throws Exception {
testTypes("/** @const */ var x = {}; x.y = null;" +
"function f(a) { x.y = a; }" +
"/** @param {string} a */ function g(a) { }" +
"function h() { g(x.y); }");
}
public void testUndeclaredGlobalProperty2() throws Exception {
testTypes("/** @const */ var x = {}; x.y = null;" +
"function f() { x.y = 3; }" +
"/** @param {string} a */ function g(a) { }" +
"function h() { g(x.y); }",
"actual parameter 1 of g does not match formal parameter\n" +
"found : (null|number)\n" +
"required: string");
}
public void testLocallyInferredGlobalProperty1() throws Exception {
// We used to have a bug where x.y.z leaked from f into h.
testTypes(
"/** @constructor */ function F() {}" +
"/** @type {number} */ F.prototype.z;" +
"/** @const */ var x = {}; /** @type {F} */ x.y;" +
"function f() { x.y.z = 'abc'; }" +
"/** @param {number} x */ function g(x) {}" +
"function h() { g(x.y.z); }",
"assignment to property z of F\n" +
"found : string\n" +
"required: number");
}
public void testPropertyInferredPropagation() throws Exception {
testTypes("/** @return {Object} */function f() { return {}; }\n" +
"function g() { var x = f(); if (x.p) x.a = 'a'; else x.a = 'b'; }\n" +
"function h() { var x = f(); x.a = false; }");
}
public void testPropertyInference1() throws Exception {
testTypes(
"/** @constructor */ function F() { this.x_ = true; }" +
"/** @return {string} */" +
"F.prototype.bar = function() { if (this.x_) return this.x_; };",
"inconsistent return type\n" +
"found : boolean\n" +
"required: string");
}
public void testPropertyInference2() throws Exception {
testTypes(
"/** @constructor */ function F() { this.x_ = true; }" +
"F.prototype.baz = function() { this.x_ = null; };" +
"/** @return {string} */" +
"F.prototype.bar = function() { if (this.x_) return this.x_; };",
"inconsistent return type\n" +
"found : boolean\n" +
"required: string");
}
public void testPropertyInference3() throws Exception {
testTypes(
"/** @constructor */ function F() { this.x_ = true; }" +
"F.prototype.baz = function() { this.x_ = 3; };" +
"/** @return {string} */" +
"F.prototype.bar = function() { if (this.x_) return this.x_; };",
"inconsistent return type\n" +
"found : (boolean|number)\n" +
"required: string");
}
public void testPropertyInference4() throws Exception {
testTypes(
"/** @constructor */ function F() { }" +
"F.prototype.x_ = 3;" +
"/** @return {string} */" +
"F.prototype.bar = function() { if (this.x_) return this.x_; };",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testPropertyInference5() throws Exception {
testTypes(
"/** @constructor */ function F() { }" +
"F.prototype.baz = function() { this.x_ = 3; };" +
"/** @return {string} */" +
"F.prototype.bar = function() { if (this.x_) return this.x_; };");
}
public void testPropertyInference6() throws Exception {
testTypes(
"/** @constructor */ function F() { }" +
"(new F).x_ = 3;" +
"/** @return {string} */" +
"F.prototype.bar = function() { return this.x_; };");
}
public void testPropertyInference7() throws Exception {
testTypes(
"/** @constructor */ function F() { this.x_ = true; }" +
"(new F).x_ = 3;" +
"/** @return {string} */" +
"F.prototype.bar = function() { return this.x_; };",
"inconsistent return type\n" +
"found : boolean\n" +
"required: string");
}
public void testPropertyInference8() throws Exception {
testTypes(
"/** @constructor */ function F() { " +
" /** @type {string} */ this.x_ = 'x';" +
"}" +
"(new F).x_ = 3;" +
"/** @return {string} */" +
"F.prototype.bar = function() { return this.x_; };",
"assignment to property x_ of F\n" +
"found : number\n" +
"required: string");
}
public void testPropertyInference9() throws Exception {
testTypes(
"/** @constructor */ function A() {}" +
"/** @return {function(): ?} */ function f() { " +
" return function() {};" +
"}" +
"var g = f();" +
"/** @type {number} */ g.prototype.bar_ = null;",
"assignment\n" +
"found : null\n" +
"required: number");
}
public void testPropertyInference10() throws Exception {
// NOTE(nicksantos): There used to be a bug where a property
// on the prototype of one structural function would leak onto
// the prototype of other variables with the same structural
// function type.
testTypes(
"/** @constructor */ function A() {}" +
"/** @return {function(): ?} */ function f() { " +
" return function() {};" +
"}" +
"var g = f();" +
"/** @type {number} */ g.prototype.bar_ = 1;" +
"var h = f();" +
"/** @type {string} */ h.prototype.bar_ = 1;",
"assignment\n" +
"found : number\n" +
"required: string");
}
public void testNoPersistentTypeInferenceForObjectProperties()
throws Exception {
testTypes("/** @param {Object} o\n@param {string} x */\n" +
"function s1(o,x) { o.x = x; }\n" +
"/** @param {Object} o\n@return {string} */\n" +
"function g1(o) { return typeof o.x == 'undefined' ? '' : o.x; }\n" +
"/** @param {Object} o\n@param {number} x */\n" +
"function s2(o,x) { o.x = x; }\n" +
"/** @param {Object} o\n@return {number} */\n" +
"function g2(o) { return typeof o.x == 'undefined' ? 0 : o.x; }");
}
public void testNoPersistentTypeInferenceForFunctionProperties()
throws Exception {
testTypes("/** @param {Function} o\n@param {string} x */\n" +
"function s1(o,x) { o.x = x; }\n" +
"/** @param {Function} o\n@return {string} */\n" +
"function g1(o) { return typeof o.x == 'undefined' ? '' : o.x; }\n" +
"/** @param {Function} o\n@param {number} x */\n" +
"function s2(o,x) { o.x = x; }\n" +
"/** @param {Function} o\n@return {number} */\n" +
"function g2(o) { return typeof o.x == 'undefined' ? 0 : o.x; }");
}
public void testObjectPropertyTypeInferredInLocalScope1() throws Exception {
testTypes("/** @param {!Object} o\n@return {string} */\n" +
"function f(o) { o.x = 1; return o.x; }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testObjectPropertyTypeInferredInLocalScope2() throws Exception {
testTypes("/**@param {!Object} o\n@param {number?} x\n@return {string}*/" +
"function f(o, x) { o.x = 'a';\nif (x) {o.x = x;}\nreturn o.x; }",
"inconsistent return type\n" +
"found : (number|string)\n" +
"required: string");
}
public void testObjectPropertyTypeInferredInLocalScope3() throws Exception {
testTypes("/**@param {!Object} o\n@param {number?} x\n@return {string}*/" +
"function f(o, x) { if (x) {o.x = x;} else {o.x = 'a';}\nreturn o.x; }",
"inconsistent return type\n" +
"found : (number|string)\n" +
"required: string");
}
public void testMismatchingOverridingInferredPropertyBeforeDeclaredProperty1()
throws Exception {
testTypes("/** @constructor */var T = function() { this.x = ''; };\n" +
"/** @type {number} */ T.prototype.x = 0;",
"assignment to property x of T\n" +
"found : string\n" +
"required: number");
}
public void testMismatchingOverridingInferredPropertyBeforeDeclaredProperty2()
throws Exception {
testTypes("/** @constructor */var T = function() { this.x = ''; };\n" +
"/** @type {number} */ T.prototype.x;",
"assignment to property x of T\n" +
"found : string\n" +
"required: number");
}
public void testMismatchingOverridingInferredPropertyBeforeDeclaredProperty3()
throws Exception {
testTypes("/** @type {Object} */ var n = {};\n" +
"/** @constructor */ n.T = function() { this.x = ''; };\n" +
"/** @type {number} */ n.T.prototype.x = 0;",
"assignment to property x of n.T\n" +
"found : string\n" +
"required: number");
}
public void testMismatchingOverridingInferredPropertyBeforeDeclaredProperty4()
throws Exception {
testTypes("var n = {};\n" +
"/** @constructor */ n.T = function() { this.x = ''; };\n" +
"/** @type {number} */ n.T.prototype.x = 0;",
"assignment to property x of n.T\n" +
"found : string\n" +
"required: number");
}
public void testPropertyUsedBeforeDefinition1() throws Exception {
testTypes("/** @constructor */ var T = function() {};\n" +
"/** @return {string} */" +
"T.prototype.f = function() { return this.g(); };\n" +
"/** @return {number} */ T.prototype.g = function() { return 1; };\n",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testPropertyUsedBeforeDefinition2() throws Exception {
testTypes("var n = {};\n" +
"/** @constructor */ n.T = function() {};\n" +
"/** @return {string} */" +
"n.T.prototype.f = function() { return this.g(); };\n" +
"/** @return {number} */ n.T.prototype.g = function() { return 1; };\n",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testAdd1() throws Exception {
testTypes("/**@return {void}*/function foo(){var a = 'abc'+foo();}");
}
public void testAdd2() throws Exception {
testTypes("/**@return {void}*/function foo(){var a = foo()+4;}");
}
public void testAdd3() throws Exception {
testTypes("/** @type {string} */ var a = 'a';" +
"/** @type {string} */ var b = 'b';" +
"/** @type {string} */ var c = a + b;");
}
public void testAdd4() throws Exception {
testTypes("/** @type {number} */ var a = 5;" +
"/** @type {string} */ var b = 'b';" +
"/** @type {string} */ var c = a + b;");
}
public void testAdd5() throws Exception {
testTypes("/** @type {string} */ var a = 'a';" +
"/** @type {number} */ var b = 5;" +
"/** @type {string} */ var c = a + b;");
}
public void testAdd6() throws Exception {
testTypes("/** @type {number} */ var a = 5;" +
"/** @type {number} */ var b = 5;" +
"/** @type {number} */ var c = a + b;");
}
public void testAdd7() throws Exception {
testTypes("/** @type {number} */ var a = 5;" +
"/** @type {string} */ var b = 'b';" +
"/** @type {number} */ var c = a + b;",
"initializing variable\n" +
"found : string\n" +
"required: number");
}
public void testAdd8() throws Exception {
testTypes("/** @type {string} */ var a = 'a';" +
"/** @type {number} */ var b = 5;" +
"/** @type {number} */ var c = a + b;",
"initializing variable\n" +
"found : string\n" +
"required: number");
}
public void testAdd9() throws Exception {
testTypes("/** @type {number} */ var a = 5;" +
"/** @type {number} */ var b = 5;" +
"/** @type {string} */ var c = a + b;",
"initializing variable\n" +
"found : number\n" +
"required: string");
}
public void testAdd10() throws Exception {
// d.e.f will have unknown type.
testTypes(
suppressMissingProperty("e", "f") +
"/** @type {number} */ var a = 5;" +
"/** @type {string} */ var c = a + d.e.f;");
}
public void testAdd11() throws Exception {
// d.e.f will have unknown type.
testTypes(
suppressMissingProperty("e", "f") +
"/** @type {number} */ var a = 5;" +
"/** @type {number} */ var c = a + d.e.f;");
}
public void testAdd12() throws Exception {
testTypes("/** @return {(number,string)} */ function a() { return 5; }" +
"/** @type {number} */ var b = 5;" +
"/** @type {boolean} */ var c = a() + b;",
"initializing variable\n" +
"found : (number|string)\n" +
"required: boolean");
}
public void testAdd13() throws Exception {
testTypes("/** @type {number} */ var a = 5;" +
"/** @return {(number,string)} */ function b() { return 5; }" +
"/** @type {boolean} */ var c = a + b();",
"initializing variable\n" +
"found : (number|string)\n" +
"required: boolean");
}
public void testAdd14() throws Exception {
testTypes("/** @type {(null,string)} */ var a = null;" +
"/** @type {number} */ var b = 5;" +
"/** @type {boolean} */ var c = a + b;",
"initializing variable\n" +
"found : (number|string)\n" +
"required: boolean");
}
public void testAdd15() throws Exception {
testTypes("/** @type {number} */ var a = 5;" +
"/** @return {(number,string)} */ function b() { return 5; }" +
"/** @type {boolean} */ var c = a + b();",
"initializing variable\n" +
"found : (number|string)\n" +
"required: boolean");
}
public void testAdd16() throws Exception {
testTypes("/** @type {(undefined,string)} */ var a = undefined;" +
"/** @type {number} */ var b = 5;" +
"/** @type {boolean} */ var c = a + b;",
"initializing variable\n" +
"found : (number|string)\n" +
"required: boolean");
}
public void testAdd17() throws Exception {
testTypes("/** @type {number} */ var a = 5;" +
"/** @type {(undefined,string)} */ var b = undefined;" +
"/** @type {boolean} */ var c = a + b;",
"initializing variable\n" +
"found : (number|string)\n" +
"required: boolean");
}
public void testAdd18() throws Exception {
testTypes("function f() {};" +
"/** @type {string} */ var a = 'a';" +
"/** @type {number} */ var c = a + f();",
"initializing variable\n" +
"found : string\n" +
"required: number");
}
public void testAdd19() throws Exception {
testTypes("/** @param {number} opt_x\n@param {number} opt_y\n" +
"@return {number} */ function f(opt_x, opt_y) {" +
"return opt_x + opt_y;}");
}
public void testAdd20() throws Exception {
testTypes("/** @param {!Number} opt_x\n@param {!Number} opt_y\n" +
"@return {number} */ function f(opt_x, opt_y) {" +
"return opt_x + opt_y;}");
}
public void testAdd21() throws Exception {
testTypes("/** @param {Number|Boolean} opt_x\n" +
"@param {number|boolean} opt_y\n" +
"@return {number} */ function f(opt_x, opt_y) {" +
"return opt_x + opt_y;}");
}
public void testNumericComparison1() throws Exception {
testTypes("/**@param {number} a*/ function f(a) {return a < 3;}");
}
public void testNumericComparison2() throws Exception {
testTypes("/**@param {!Object} a*/ function f(a) {return a < 3;}",
"left side of numeric comparison\n" +
"found : Object\n" +
"required: number");
}
public void testNumericComparison3() throws Exception {
testTypes("/**@param {string} a*/ function f(a) {return a < 3;}");
}
public void testNumericComparison4() throws Exception {
testTypes("/**@param {(number,undefined)} a*/ " +
"function f(a) {return a < 3;}");
}
public void testNumericComparison5() throws Exception {
testTypes("/**@param {*} a*/ function f(a) {return a < 3;}",
"left side of numeric comparison\n" +
"found : *\n" +
"required: number");
}
public void testNumericComparison6() throws Exception {
testTypes("/**@return {void} */ function foo() { if (3 >= foo()) return; }",
"right side of numeric comparison\n" +
"found : undefined\n" +
"required: number");
}
public void testStringComparison1() throws Exception {
testTypes("/**@param {string} a*/ function f(a) {return a < 'x';}");
}
public void testStringComparison2() throws Exception {
testTypes("/**@param {Object} a*/ function f(a) {return a < 'x';}");
}
public void testStringComparison3() throws Exception {
testTypes("/**@param {number} a*/ function f(a) {return a < 'x';}");
}
public void testStringComparison4() throws Exception {
testTypes("/**@param {string|undefined} a*/ " +
"function f(a) {return a < 'x';}");
}
public void testStringComparison5() throws Exception {
testTypes("/**@param {*} a*/ " +
"function f(a) {return a < 'x';}");
}
public void testStringComparison6() throws Exception {
testTypes("/**@return {void} */ " +
"function foo() { if ('a' >= foo()) return; }",
"right side of comparison\n" +
"found : undefined\n" +
"required: string");
}
public void testValueOfComparison1() throws Exception {
testTypes("/** @constructor */function O() {};" +
"/**@override*/O.prototype.valueOf = function() { return 1; };" +
"/**@param {!O} a\n@param {!O} b*/ function f(a,b) { return a < b; }");
}
public void testValueOfComparison2() throws Exception {
testTypes("/** @constructor */function O() {};" +
"/**@override*/O.prototype.valueOf = function() { return 1; };" +
"/**@param {!O} a\n@param {number} b*/" +
"function f(a,b) { return a < b; }");
}
public void testValueOfComparison3() throws Exception {
testTypes("/** @constructor */function O() {};" +
"/**@override*/O.prototype.toString = function() { return 'o'; };" +
"/**@param {!O} a\n@param {string} b*/" +
"function f(a,b) { return a < b; }");
}
public void testGenericRelationalExpression() throws Exception {
testTypes("/**@param {*} a\n@param {*} b*/ " +
"function f(a,b) {return a < b;}");
}
public void testInstanceof1() throws Exception {
testTypes("function foo(){" +
"if (bar instanceof 3)return;}",
"instanceof requires an object\n" +
"found : number\n" +
"required: Object");
}
public void testInstanceof2() throws Exception {
testTypes("/**@return {void}*/function foo(){" +
"if (foo() instanceof Object)return;}",
"deterministic instanceof yields false\n" +
"found : undefined\n" +
"required: NoObject");
}
public void testInstanceof3() throws Exception {
testTypes("/**@return {*} */function foo(){" +
"if (foo() instanceof Object)return;}");
}
public void testInstanceof4() throws Exception {
testTypes("/**@return {(Object|number)} */function foo(){" +
"if (foo() instanceof Object)return 3;}");
}
public void testInstanceof5() throws Exception {
// No warning for unknown types.
testTypes("/** @return {?} */ function foo(){" +
"if (foo() instanceof Object)return;}");
}
public void testInstanceof6() throws Exception {
testTypes("/**@return {(Array|number)} */function foo(){" +
"if (foo() instanceof Object)return 3;}");
}
public void testInstanceOfReduction3() throws Exception {
testTypes(
"/** \n" +
" * @param {Object} x \n" +
" * @param {Function} y \n" +
" * @return {boolean} \n" +
" */\n" +
"var f = function(x, y) {\n" +
" return x instanceof y;\n" +
"};");
}
public void testScoping1() throws Exception {
testTypes(
"/**@param {string} a*/function foo(a){" +
" /**@param {Array|string} a*/function bar(a){" +
" if (a instanceof Array)return;" +
" }" +
"}");
}
public void testScoping2() throws Exception {
testTypes(
"/** @type number */ var a;" +
"function Foo() {" +
" /** @type string */ var a;" +
"}");
}
public void testScoping3() throws Exception {
testTypes("\n\n/** @type{Number}*/var b;\n/** @type{!String} */var b;",
"variable b redefined with type String, original " +
"definition at [testcode]:3 with type (Number|null)");
}
public void testScoping4() throws Exception {
testTypes("/** @type{Number}*/var b; if (true) /** @type{!String} */var b;",
"variable b redefined with type String, original " +
"definition at [testcode]:1 with type (Number|null)");
}
public void testScoping5() throws Exception {
// multiple definitions are not checked by the type checker but by a
// subsequent pass
testTypes("if (true) var b; var b;");
}
public void testScoping6() throws Exception {
// multiple definitions are not checked by the type checker but by a
// subsequent pass
testTypes("if (true) var b; if (true) var b;");
}
public void testScoping7() throws Exception {
testTypes("/** @constructor */function A() {" +
" /** @type !A */this.a = null;" +
"}",
"assignment to property a of A\n" +
"found : null\n" +
"required: A");
}
public void testScoping8() throws Exception {
testTypes("/** @constructor */function A() {}" +
"/** @constructor */function B() {" +
" /** @type !A */this.a = null;" +
"}",
"assignment to property a of B\n" +
"found : null\n" +
"required: A");
}
public void testScoping9() throws Exception {
testTypes("/** @constructor */function B() {" +
" /** @type !A */this.a = null;" +
"}" +
"/** @constructor */function A() {}",
"assignment to property a of B\n" +
"found : null\n" +
"required: A");
}
public void testScoping10() throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope("var a = function b(){};");
// a declared, b is not
assertTrue(p.scope.isDeclared("a", false));
assertFalse(p.scope.isDeclared("b", false));
// checking that a has the correct assigned type
assertEquals("function (): undefined",
p.scope.getVar("a").getType().toString());
}
public void testScoping11() throws Exception {
// named function expressions create a binding in their body only
// the return is wrong but the assignment is ok since the type of b is ?
testTypes(
"/** @return {number} */var a = function b(){ return b };",
"inconsistent return type\n" +
"found : function (): number\n" +
"required: number");
}
public void testScoping12() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** @type {number} */ F.prototype.bar = 3;" +
"/** @param {!F} f */ function g(f) {" +
" /** @return {string} */" +
" function h() {" +
" return f.bar;" +
" }" +
"}",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testFunctionArguments1() throws Exception {
testFunctionType(
"/** @param {number} a\n@return {string} */" +
"function f(a) {}",
"function (number): string");
}
public void testFunctionArguments2() throws Exception {
testFunctionType(
"/** @param {number} opt_a\n@return {string} */" +
"function f(opt_a) {}",
"function (number=): string");
}
public void testFunctionArguments3() throws Exception {
testFunctionType(
"/** @param {number} b\n@return {string} */" +
"function f(a,b) {}",
"function (?, number): string");
}
public void testFunctionArguments4() throws Exception {
testFunctionType(
"/** @param {number} opt_a\n@return {string} */" +
"function f(a,opt_a) {}",
"function (?, number=): string");
}
public void testFunctionArguments5() throws Exception {
testTypes(
"function a(opt_a,a) {}",
"optional arguments must be at the end");
}
public void testFunctionArguments6() throws Exception {
testTypes(
"function a(var_args,a) {}",
"variable length argument must be last");
}
public void testFunctionArguments7() throws Exception {
testTypes(
"/** @param {number} opt_a\n@return {string} */" +
"function a(a,opt_a,var_args) {}");
}
public void testFunctionArguments8() throws Exception {
testTypes(
"function a(a,opt_a,var_args,b) {}",
"variable length argument must be last");
}
public void testFunctionArguments9() throws Exception {
// testing that only one error is reported
testTypes(
"function a(a,opt_a,var_args,b,c) {}",
"variable length argument must be last");
}
public void testFunctionArguments10() throws Exception {
// testing that only one error is reported
testTypes(
"function a(a,opt_a,b,c) {}",
"optional arguments must be at the end");
}
public void testFunctionArguments11() throws Exception {
testTypes(
"function a(a,opt_a,b,c,var_args,d) {}",
"optional arguments must be at the end");
}
public void testFunctionArguments12() throws Exception {
testTypes("/** @param foo {String} */function bar(baz){}",
"parameter foo does not appear in bar's parameter list");
}
public void testFunctionArguments13() throws Exception {
// verifying that the argument type have non-inferrable types
testTypes(
"/** @return {boolean} */ function u() { return true; }" +
"/** @param {boolean} b\n@return {?boolean} */" +
"function f(b) { if (u()) { b = null; } return b; }",
"assignment\n" +
"found : null\n" +
"required: boolean");
}
public void testFunctionArguments14() throws Exception {
testTypes(
"/**\n" +
" * @param {string} x\n" +
" * @param {number} opt_y\n" +
" * @param {boolean} var_args\n" +
" */ function f(x, opt_y, var_args) {}" +
"f('3'); f('3', 2); f('3', 2, true); f('3', 2, true, false);");
}
public void testFunctionArguments15() throws Exception {
testTypes(
"/** @param {?function(*)} f */" +
"function g(f) { f(1, 2); }",
"Function f: called with 2 argument(s). " +
"Function requires at least 1 argument(s) " +
"and no more than 1 argument(s).");
}
public void testFunctionArguments16() throws Exception {
testTypes(
"/** @param {...number} var_args */" +
"function g(var_args) {} g(1, true);",
"actual parameter 2 of g does not match formal parameter\n" +
"found : boolean\n" +
"required: (number|undefined)");
}
public void testPrintFunctionName1() throws Exception {
// Ensures that the function name is pretty.
testTypes(
"var goog = {}; goog.run = function(f) {};" +
"goog.run();",
"Function goog.run: called with 0 argument(s). " +
"Function requires at least 1 argument(s) " +
"and no more than 1 argument(s).");
}
public void testPrintFunctionName2() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {}; " +
"Foo.prototype.run = function(f) {};" +
"(new Foo).run();",
"Function Foo.prototype.run: called with 0 argument(s). " +
"Function requires at least 1 argument(s) " +
"and no more than 1 argument(s).");
}
public void testFunctionInference1() throws Exception {
testFunctionType(
"function f(a) {}",
"function (?): undefined");
}
public void testFunctionInference2() throws Exception {
testFunctionType(
"function f(a,b) {}",
"function (?, ?): undefined");
}
public void testFunctionInference3() throws Exception {
testFunctionType(
"function f(var_args) {}",
"function (...[?]): undefined");
}
public void testFunctionInference4() throws Exception {
testFunctionType(
"function f(a,b,c,var_args) {}",
"function (?, ?, ?, ...[?]): undefined");
}
public void testFunctionInference5() throws Exception {
testFunctionType(
"/** @this Date\n@return {string} */function f(a) {}",
"function (this:Date, ?): string");
}
public void testFunctionInference6() throws Exception {
testFunctionType(
"/** @this Date\n@return {string} */function f(opt_a) {}",
"function (this:Date, ?=): string");
}
public void testFunctionInference7() throws Exception {
testFunctionType(
"/** @this Date */function f(a,b,c,var_args) {}",
"function (this:Date, ?, ?, ?, ...[?]): undefined");
}
public void testFunctionInference8() throws Exception {
testFunctionType(
"function f() {}",
"function (): undefined");
}
public void testFunctionInference9() throws Exception {
testFunctionType(
"var f = function() {};",
"function (): undefined");
}
public void testFunctionInference10() throws Exception {
testFunctionType(
"/** @this Date\n@param {boolean} b\n@return {string} */" +
"var f = function(a,b) {};",
"function (this:Date, ?, boolean): string");
}
public void testFunctionInference11() throws Exception {
testFunctionType(
"var goog = {};" +
"/** @return {number}*/goog.f = function(){};",
"goog.f",
"function (): number");
}
public void testFunctionInference12() throws Exception {
testFunctionType(
"var goog = {};" +
"goog.f = function(){};",
"goog.f",
"function (): undefined");
}
public void testFunctionInference13() throws Exception {
testFunctionType(
"var goog = {};" +
"/** @constructor */ goog.Foo = function(){};" +
"/** @param {!goog.Foo} f */function eatFoo(f){};",
"eatFoo",
"function (goog.Foo): undefined");
}
public void testFunctionInference14() throws Exception {
testFunctionType(
"var goog = {};" +
"/** @constructor */ goog.Foo = function(){};" +
"/** @return {!goog.Foo} */function eatFoo(){ return new goog.Foo; };",
"eatFoo",
"function (): goog.Foo");
}
public void testFunctionInference15() throws Exception {
testFunctionType(
"/** @constructor */ function f() {};" +
"f.prototype.foo = function(){};",
"f.prototype.foo",
"function (this:f): undefined");
}
public void testFunctionInference16() throws Exception {
testFunctionType(
"/** @constructor */ function f() {};" +
"f.prototype.foo = function(){};",
"(new f).foo",
"function (this:f): undefined");
}
public void testFunctionInference17() throws Exception {
testFunctionType(
"/** @constructor */ function f() {}" +
"function abstractMethod() {}" +
"/** @param {number} x */ f.prototype.foo = abstractMethod;",
"(new f).foo",
"function (this:f, number): ?");
}
public void testFunctionInference18() throws Exception {
testFunctionType(
"var goog = {};" +
"/** @this {Date} */ goog.eatWithDate;",
"goog.eatWithDate",
"function (this:Date): ?");
}
public void testFunctionInference19() throws Exception {
testFunctionType(
"/** @param {string} x */ var f;",
"f",
"function (string): ?");
}
public void testFunctionInference20() throws Exception {
testFunctionType(
"/** @this {Date} */ var f;",
"f",
"function (this:Date): ?");
}
public void testInnerFunction1() throws Exception {
testTypes(
"function f() {" +
" /** @type {number} */ var x = 3;\n" +
" function g() { x = null; }" +
" return x;" +
"}",
"assignment\n" +
"found : null\n" +
"required: number");
}
public void testInnerFunction2() throws Exception {
testTypes(
"/** @return {number} */\n" +
"function f() {" +
" var x = null;\n" +
" function g() { x = 3; }" +
" g();" +
" return x;" +
"}",
"inconsistent return type\n" +
"found : (null|number)\n" +
"required: number");
}
public void testInnerFunction3() throws Exception {
testTypes(
"var x = null;" +
"/** @return {number} */\n" +
"function f() {" +
" x = 3;\n" +
" /** @return {number} */\n" +
" function g() { x = true; return x; }" +
" return x;" +
"}",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testInnerFunction4() throws Exception {
testTypes(
"var x = null;" +
"/** @return {number} */\n" +
"function f() {" +
" x = '3';\n" +
" /** @return {number} */\n" +
" function g() { x = 3; return x; }" +
" return x;" +
"}",
"inconsistent return type\n" +
"found : string\n" +
"required: number");
}
public void testInnerFunction5() throws Exception {
testTypes(
"/** @return {number} */\n" +
"function f() {" +
" var x = 3;\n" +
" /** @return {number} */" +
" function g() { var x = 3;x = true; return x; }" +
" return x;" +
"}",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testInnerFunction6() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"function f() {" +
" var x = 0 || function() {};\n" +
" function g() { if (goog.isFunction(x)) { x(1); } }" +
" g();" +
"}", null);
}
public void testInnerFunction7() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"function f() {" +
" /** @type {number|function()} */" +
" var x = 0 || function() {};\n" +
" function g() { if (goog.isFunction(x)) { x(1); } }" +
" g();" +
"}",
"Function x: called with 1 argument(s). " +
"Function requires at least 0 argument(s) " +
"and no more than 0 argument(s).");
}
public void testInnerFunction8() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"function f() {" +
" function x() {};\n" +
" function g() { if (goog.isFunction(x)) { x(1); } }" +
" g();" +
"}",
"Function x: called with 1 argument(s). " +
"Function requires at least 0 argument(s) " +
"and no more than 0 argument(s).");
}
public void testInnerFunction9() throws Exception {
testTypes(
"function f() {" +
" var x = 3;\n" +
" function g() { x = null; };\n" +
" function h() { return x == null; }" +
" return h();" +
"}");
}
public void testInnerFunction10() throws Exception {
testTypes(
"function f() {" +
" /** @type {?number} */ var x = null;" +
" /** @return {string} */" +
" function g() {" +
" if (!x) {" +
" x = 1;" +
" }" +
" return x;" +
" }" +
"}",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testInnerFunction11() throws Exception {
// TODO(nicksantos): This is actually bad inference, because
// h sets x to null. We should fix this, but for now we do it
// this way so that we don't break existing binaries. We will
// need to change TypeInference#isUnflowable to fix this.
testTypes(
"function f() {" +
" /** @type {?number} */ var x = null;" +
" /** @return {number} */" +
" function g() {" +
" x = 1;" +
" h();" +
" return x;" +
" }" +
" function h() {" +
" x = null;" +
" }" +
"}");
}
public void testAbstractMethodHandling1() throws Exception {
testTypes(
"/** @type {Function} */ var abstractFn = function() {};" +
"abstractFn(1);");
}
public void testAbstractMethodHandling2() throws Exception {
testTypes(
"var abstractFn = function() {};" +
"abstractFn(1);",
"Function abstractFn: called with 1 argument(s). " +
"Function requires at least 0 argument(s) " +
"and no more than 0 argument(s).");
}
public void testAbstractMethodHandling3() throws Exception {
testTypes(
"var goog = {};" +
"/** @type {Function} */ goog.abstractFn = function() {};" +
"goog.abstractFn(1);");
}
public void testAbstractMethodHandling4() throws Exception {
testTypes(
"var goog = {};" +
"goog.abstractFn = function() {};" +
"goog.abstractFn(1);",
"Function goog.abstractFn: called with 1 argument(s). " +
"Function requires at least 0 argument(s) " +
"and no more than 0 argument(s).");
}
public void testAbstractMethodHandling5() throws Exception {
testTypes(
"/** @type {!Function} */ var abstractFn = function() {};" +
"/** @param {number} x */ var f = abstractFn;" +
"f('x');",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testAbstractMethodHandling6() throws Exception {
testTypes(
"var goog = {};" +
"/** @type {Function} */ goog.abstractFn = function() {};" +
"/** @param {number} x */ goog.f = abstractFn;" +
"goog.f('x');",
"actual parameter 1 of goog.f does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testMethodInference1() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** @return {number} */ F.prototype.foo = function() { return 3; };" +
"/** @constructor \n * @extends {F} */ " +
"function G() {}" +
"/** @override */ G.prototype.foo = function() { return true; };",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testMethodInference2() throws Exception {
testTypes(
"var goog = {};" +
"/** @constructor */ goog.F = function() {};" +
"/** @return {number} */ goog.F.prototype.foo = " +
" function() { return 3; };" +
"/** @constructor \n * @extends {goog.F} */ " +
"goog.G = function() {};" +
"/** @override */ goog.G.prototype.foo = function() { return true; };",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testMethodInference3() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** @param {boolean} x \n * @return {number} */ " +
"F.prototype.foo = function(x) { return 3; };" +
"/** @constructor \n * @extends {F} */ " +
"function G() {}" +
"/** @override */ " +
"G.prototype.foo = function(x) { return x; };",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testMethodInference4() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** @param {boolean} x \n * @return {number} */ " +
"F.prototype.foo = function(x) { return 3; };" +
"/** @constructor \n * @extends {F} */ " +
"function G() {}" +
"/** @override */ " +
"G.prototype.foo = function(y) { return y; };",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testMethodInference5() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** @param {number} x \n * @return {string} */ " +
"F.prototype.foo = function(x) { return 'x'; };" +
"/** @constructor \n * @extends {F} */ " +
"function G() {}" +
"/** @type {number} */ G.prototype.num = 3;" +
"/** @override */ " +
"G.prototype.foo = function(y) { return this.num + y; };",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testMethodInference6() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** @param {number} x */ F.prototype.foo = function(x) { };" +
"/** @constructor \n * @extends {F} */ " +
"function G() {}" +
"/** @override */ G.prototype.foo = function() { };" +
"(new G()).foo(1);");
}
public void testMethodInference7() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"F.prototype.foo = function() { };" +
"/** @constructor \n * @extends {F} */ " +
"function G() {}" +
"/** @override */ G.prototype.foo = function(x, y) { };",
"mismatch of the foo property type and the type of the property " +
"it overrides from superclass F\n" +
"original: function (this:F): undefined\n" +
"override: function (this:G, ?, ?): undefined");
}
public void testMethodInference8() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"F.prototype.foo = function() { };" +
"/** @constructor \n * @extends {F} */ " +
"function G() {}" +
"/** @override */ " +
"G.prototype.foo = function(opt_b, var_args) { };" +
"(new G()).foo(1, 2, 3);");
}
public void testMethodInference9() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"F.prototype.foo = function() { };" +
"/** @constructor \n * @extends {F} */ " +
"function G() {}" +
"/** @override */ " +
"G.prototype.foo = function(var_args, opt_b) { };",
"variable length argument must be last");
}
public void testStaticMethodDeclaration1() throws Exception {
testTypes(
"/** @constructor */ function F() { F.foo(true); }" +
"/** @param {number} x */ F.foo = function(x) {};",
"actual parameter 1 of F.foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testStaticMethodDeclaration2() throws Exception {
testTypes(
"var goog = goog || {}; function f() { goog.foo(true); }" +
"/** @param {number} x */ goog.foo = function(x) {};",
"actual parameter 1 of goog.foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testStaticMethodDeclaration3() throws Exception {
testTypes(
"var goog = goog || {}; function f() { goog.foo(true); }" +
"goog.foo = function() {};",
"Function goog.foo: called with 1 argument(s). Function requires " +
"at least 0 argument(s) and no more than 0 argument(s).");
}
public void testDuplicateStaticMethodDecl1() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @param {number} x */ goog.foo = function(x) {};" +
"/** @param {number} x */ goog.foo = function(x) {};",
"variable goog.foo redefined with type function (number): undefined, " +
"original definition at [testcode]:1 " +
"with type function (number): undefined");
}
public void testDuplicateStaticMethodDecl2() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @param {number} x */ goog.foo = function(x) {};" +
"/** @param {number} x \n * @suppress {duplicate} */ " +
"goog.foo = function(x) {};");
}
public void testDuplicateStaticMethodDecl3() throws Exception {
testTypes(
"var goog = goog || {};" +
"goog.foo = function(x) {};" +
"goog.foo = function(x) {};");
}
public void testDuplicateStaticMethodDecl4() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @type {Function} */ goog.foo = function(x) {};" +
"goog.foo = function(x) {};");
}
public void testDuplicateStaticMethodDecl5() throws Exception {
testTypes(
"var goog = goog || {};" +
"goog.foo = function(x) {};" +
"/** @return {undefined} */ goog.foo = function(x) {};",
"variable goog.foo redefined with type function (?): undefined, " +
"original definition at [testcode]:1 with type " +
"function (?): undefined");
}
public void testDuplicateStaticPropertyDecl1() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @type {Foo} */ goog.foo;" +
"/** @type {Foo} */ goog.foo;" +
"/** @constructor */ function Foo() {}");
}
public void testDuplicateStaticPropertyDecl2() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @type {Foo} */ goog.foo;" +
"/** @type {Foo} \n * @suppress {duplicate} */ goog.foo;" +
"/** @constructor */ function Foo() {}");
}
public void testDuplicateStaticPropertyDecl3() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @type {!Foo} */ goog.foo;" +
"/** @type {string} */ goog.foo;" +
"/** @constructor */ function Foo() {}",
"variable goog.foo redefined with type string, " +
"original definition at [testcode]:1 with type Foo");
}
public void testDuplicateStaticPropertyDecl4() throws Exception {
testClosureTypesMultipleWarnings(
"var goog = goog || {};" +
"/** @type {!Foo} */ goog.foo;" +
"/** @type {string} */ goog.foo = 'x';" +
"/** @constructor */ function Foo() {}",
Lists.newArrayList(
"assignment to property foo of goog\n" +
"found : string\n" +
"required: Foo",
"variable goog.foo redefined with type string, " +
"original definition at [testcode]:1 with type Foo"));
}
public void testDuplicateStaticPropertyDecl5() throws Exception {
testClosureTypesMultipleWarnings(
"var goog = goog || {};" +
"/** @type {!Foo} */ goog.foo;" +
"/** @type {string}\n * @suppress {duplicate} */ goog.foo = 'x';" +
"/** @constructor */ function Foo() {}",
Lists.newArrayList(
"assignment to property foo of goog\n" +
"found : string\n" +
"required: Foo",
"variable goog.foo redefined with type string, " +
"original definition at [testcode]:1 with type Foo"));
}
public void testDuplicateStaticPropertyDecl6() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @type {string} */ goog.foo = 'y';" +
"/** @type {string}\n * @suppress {duplicate} */ goog.foo = 'x';");
}
public void testDuplicateStaticPropertyDecl7() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @param {string} x */ goog.foo;" +
"/** @type {function(string)} */ goog.foo;");
}
public void testDuplicateStaticPropertyDecl8() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @return {EventCopy} */ goog.foo;" +
"/** @constructor */ function EventCopy() {}" +
"/** @return {EventCopy} */ goog.foo;");
}
public void testDuplicateStaticPropertyDecl9() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @return {EventCopy} */ goog.foo;" +
"/** @return {EventCopy} */ goog.foo;" +
"/** @constructor */ function EventCopy() {}");
}
public void testDuplicateStaticPropertyDec20() throws Exception {
testTypes(
"/**\n" +
" * @fileoverview\n" +
" * @suppress {duplicate}\n" +
" */" +
"var goog = goog || {};" +
"/** @type {string} */ goog.foo = 'y';" +
"/** @type {string} */ goog.foo = 'x';");
}
public void testDuplicateLocalVarDecl() throws Exception {
testClosureTypesMultipleWarnings(
"/** @param {number} x */\n" +
"function f(x) { /** @type {string} */ var x = ''; }",
Lists.newArrayList(
"variable x redefined with type string, original definition" +
" at [testcode]:2 with type number",
"initializing variable\n" +
"found : string\n" +
"required: number"));
}
public void testDuplicateInstanceMethod1() throws Exception {
// If there's no jsdoc on the methods, then we treat them like
// any other inferred properties.
testTypes(
"/** @constructor */ function F() {}" +
"F.prototype.bar = function() {};" +
"F.prototype.bar = function() {};");
}
public void testDuplicateInstanceMethod2() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** jsdoc */ F.prototype.bar = function() {};" +
"/** jsdoc */ F.prototype.bar = function() {};",
"variable F.prototype.bar redefined with type " +
"function (this:F): undefined, original definition at " +
"[testcode]:1 with type function (this:F): undefined");
}
public void testDuplicateInstanceMethod3() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"F.prototype.bar = function() {};" +
"/** jsdoc */ F.prototype.bar = function() {};",
"variable F.prototype.bar redefined with type " +
"function (this:F): undefined, original definition at " +
"[testcode]:1 with type function (this:F): undefined");
}
public void testDuplicateInstanceMethod4() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** jsdoc */ F.prototype.bar = function() {};" +
"F.prototype.bar = function() {};");
}
public void testDuplicateInstanceMethod5() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** jsdoc \n * @return {number} */ F.prototype.bar = function() {" +
" return 3;" +
"};" +
"/** jsdoc \n * @suppress {duplicate} */ " +
"F.prototype.bar = function() { return ''; };",
"inconsistent return type\n" +
"found : string\n" +
"required: number");
}
public void testDuplicateInstanceMethod6() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** jsdoc \n * @return {number} */ F.prototype.bar = function() {" +
" return 3;" +
"};" +
"/** jsdoc \n * @return {string} * \n @suppress {duplicate} */ " +
"F.prototype.bar = function() { return ''; };",
"assignment to property bar of F.prototype\n" +
"found : function (this:F): string\n" +
"required: function (this:F): number");
}
public void testStubFunctionDeclaration1() throws Exception {
testFunctionType(
"/** @constructor */ function f() {};" +
"/** @param {number} x \n * @param {string} y \n" +
" * @return {number} */ f.prototype.foo;",
"(new f).foo",
"function (this:f, number, string): number");
}
public void testStubFunctionDeclaration2() throws Exception {
testExternFunctionType(
// externs
"/** @constructor */ function f() {};" +
"/** @constructor \n * @extends {f} */ f.subclass;",
"f.subclass",
"function (new:f.subclass): ?");
}
public void testStubFunctionDeclaration3() throws Exception {
testFunctionType(
"/** @constructor */ function f() {};" +
"/** @return {undefined} */ f.foo;",
"f.foo",
"function (): undefined");
}
public void testStubFunctionDeclaration4() throws Exception {
testFunctionType(
"/** @constructor */ function f() { " +
" /** @return {number} */ this.foo;" +
"}",
"(new f).foo",
"function (this:f): number");
}
public void testStubFunctionDeclaration5() throws Exception {
testFunctionType(
"/** @constructor */ function f() { " +
" /** @type {Function} */ this.foo;" +
"}",
"(new f).foo",
createNullableType(U2U_CONSTRUCTOR_TYPE).toString());
}
public void testStubFunctionDeclaration6() throws Exception {
testFunctionType(
"/** @constructor */ function f() {} " +
"/** @type {Function} */ f.prototype.foo;",
"(new f).foo",
createNullableType(U2U_CONSTRUCTOR_TYPE).toString());
}
public void testStubFunctionDeclaration7() throws Exception {
testFunctionType(
"/** @constructor */ function f() {} " +
"/** @type {Function} */ f.prototype.foo = function() {};",
"(new f).foo",
createNullableType(U2U_CONSTRUCTOR_TYPE).toString());
}
public void testStubFunctionDeclaration8() throws Exception {
testFunctionType(
"/** @type {Function} */ var f = function() {}; ",
"f",
createNullableType(U2U_CONSTRUCTOR_TYPE).toString());
}
public void testStubFunctionDeclaration9() throws Exception {
testFunctionType(
"/** @type {function():number} */ var f; ",
"f",
"function (): number");
}
public void testStubFunctionDeclaration10() throws Exception {
testFunctionType(
"/** @type {function(number):number} */ var f = function(x) {};",
"f",
"function (number): number");
}
public void testNestedFunctionInference1() throws Exception {
String nestedAssignOfFooAndBar =
"/** @constructor */ function f() {};" +
"f.prototype.foo = f.prototype.bar = function(){};";
testFunctionType(nestedAssignOfFooAndBar, "(new f).bar",
"function (this:f): undefined");
}
/**
* Tests the type of a function definition. The function defined by
* {@code functionDef} should be named {@code "f"}.
*/
private void testFunctionType(String functionDef, String functionType)
throws Exception {
testFunctionType(functionDef, "f", functionType);
}
/**
* Tests the type of a function definition. The function defined by
* {@code functionDef} should be named {@code functionName}.
*/
private void testFunctionType(String functionDef, String functionName,
String functionType) throws Exception {
// using the variable initialization check to verify the function's type
testTypes(
functionDef +
"/** @type number */var a=" + functionName + ";",
"initializing variable\n" +
"found : " + functionType + "\n" +
"required: number");
}
/**
* Tests the type of a function definition in externs.
* The function defined by {@code functionDef} should be
* named {@code functionName}.
*/
private void testExternFunctionType(String functionDef, String functionName,
String functionType) throws Exception {
testTypes(
functionDef,
"/** @type number */var a=" + functionName + ";",
"initializing variable\n" +
"found : " + functionType + "\n" +
"required: number", false);
}
public void testTypeRedefinition() throws Exception {
testTypes("a={};/**@enum {string}*/ a.A = {ZOR:'b'};"
+ "/** @constructor */ a.A = function() {}",
"variable a.A redefined with type function (new:a.A): undefined, " +
"original definition at [testcode]:1 with type enum{a.A}");
}
public void testIn1() throws Exception {
testTypes("'foo' in Object");
}
public void testIn2() throws Exception {
testTypes("3 in Object");
}
public void testIn3() throws Exception {
testTypes("undefined in Object");
}
public void testIn4() throws Exception {
testTypes("Date in Object",
"left side of 'in'\n" +
"found : function (new:Date, ?=, ?=, ?=, ?=, ?=, ?=, ?=): string\n" +
"required: string");
}
public void testIn5() throws Exception {
testTypes("'x' in null",
"'in' requires an object\n" +
"found : null\n" +
"required: Object");
}
public void testIn6() throws Exception {
testTypes(
"/** @param {number} x */" +
"function g(x) {}" +
"g(1 in {});",
"actual parameter 1 of g does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testIn7() throws Exception {
// Make sure we do inference in the 'in' expression.
testTypes(
"/**\n" +
" * @param {number} x\n" +
" * @return {number}\n" +
" */\n" +
"function g(x) { return 5; }" +
"function f() {" +
" var x = {};" +
" x.foo = '3';" +
" return g(x.foo) in {};" +
"}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testForIn1() throws Exception {
testTypes(
"/** @param {boolean} x */ function f(x) {}" +
"for (var k in {}) {" +
" f(k);" +
"}",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: boolean");
}
public void testForIn2() throws Exception {
testTypes(
"/** @param {boolean} x */ function f(x) {}" +
"/** @enum {string} */ var E = {FOO: 'bar'};" +
"/** @type {Object.<E, string>} */ var obj = {};" +
"var k = null;" +
"for (k in obj) {" +
" f(k);" +
"}",
"actual parameter 1 of f does not match formal parameter\n" +
"found : E.<string>\n" +
"required: boolean");
}
public void testForIn3() throws Exception {
testTypes(
"/** @param {boolean} x */ function f(x) {}" +
"/** @type {Object.<number>} */ var obj = {};" +
"for (var k in obj) {" +
" f(obj[k]);" +
"}",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: boolean");
}
public void testForIn4() throws Exception {
testTypes(
"/** @param {boolean} x */ function f(x) {}" +
"/** @enum {string} */ var E = {FOO: 'bar'};" +
"/** @type {Object.<E, Array>} */ var obj = {};" +
"for (var k in obj) {" +
" f(obj[k]);" +
"}",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (Array|null)\n" +
"required: boolean");
}
public void testForIn5() throws Exception {
testTypes(
"/** @param {boolean} x */ function f(x) {}" +
"/** @constructor */ var E = function(){};" +
"/** @type {Object.<E, number>} */ var obj = {};" +
"for (var k in obj) {" +
" f(k);" +
"}",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: boolean");
}
// TODO(nicksantos): change this to something that makes sense.
// public void testComparison1() throws Exception {
// testTypes("/**@type null */var a;" +
// "/**@type !Date */var b;" +
// "if (a==b) {}",
// "condition always evaluates to false\n" +
// "left : null\n" +
// "right: Date");
// }
public void testComparison2() throws Exception {
testTypes("/**@type number*/var a;" +
"/**@type !Date */var b;" +
"if (a!==b) {}",
"condition always evaluates to the same value\n" +
"left : number\n" +
"right: Date");
}
public void testComparison3() throws Exception {
// Since null == undefined in JavaScript, this code is reasonable.
testTypes("/** @type {(Object,undefined)} */var a;" +
"var b = a == null");
}
public void testComparison4() throws Exception {
testTypes("/** @type {(!Object,undefined)} */var a;" +
"/** @type {!Object} */var b;" +
"var c = a == b");
}
public void testComparison5() throws Exception {
testTypes("/** @type null */var a;" +
"/** @type null */var b;" +
"a == b",
"condition always evaluates to true\n" +
"left : null\n" +
"right: null");
}
public void testComparison6() throws Exception {
testTypes("/** @type null */var a;" +
"/** @type null */var b;" +
"a != b",
"condition always evaluates to false\n" +
"left : null\n" +
"right: null");
}
public void testComparison7() throws Exception {
testTypes("var a;" +
"var b;" +
"a == b",
"condition always evaluates to true\n" +
"left : undefined\n" +
"right: undefined");
}
public void testComparison8() throws Exception {
testTypes("/** @type {Array.<string>} */ var a = [];" +
"a[0] == null || a[1] == undefined");
}
public void testComparison9() throws Exception {
testTypes("/** @type {Array.<undefined>} */ var a = [];" +
"a[0] == null",
"condition always evaluates to true\n" +
"left : undefined\n" +
"right: null");
}
public void testComparison10() throws Exception {
testTypes("/** @type {Array.<undefined>} */ var a = [];" +
"a[0] === null");
}
public void testComparison11() throws Exception {
testTypes(
"(function(){}) == 'x'",
"condition always evaluates to false\n" +
"left : function (): undefined\n" +
"right: string");
}
public void testComparison12() throws Exception {
testTypes(
"(function(){}) == 3",
"condition always evaluates to false\n" +
"left : function (): undefined\n" +
"right: number");
}
public void testComparison13() throws Exception {
testTypes(
"(function(){}) == false",
"condition always evaluates to false\n" +
"left : function (): undefined\n" +
"right: boolean");
}
public void testDeleteOperator1() throws Exception {
testTypes(
"var x = {};" +
"/** @return {string} */ function f() { return delete x['a']; }",
"inconsistent return type\n" +
"found : boolean\n" +
"required: string");
}
public void testDeleteOperator2() throws Exception {
testTypes(
"var obj = {};" +
"/** \n" +
" * @param {string} x\n" +
" * @return {Object} */ function f(x) { return obj; }" +
"/** @param {?number} x */ function g(x) {" +
" if (x) { delete f(x)['a']; }" +
"}",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testEnumStaticMethod1() throws Exception {
testTypes(
"/** @enum */ var Foo = {AAA: 1};" +
"/** @param {number} x */ Foo.method = function(x) {};" +
"Foo.method(true);",
"actual parameter 1 of Foo.method does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testEnumStaticMethod2() throws Exception {
testTypes(
"/** @enum */ var Foo = {AAA: 1};" +
"/** @param {number} x */ Foo.method = function(x) {};" +
"function f() { Foo.method(true); }",
"actual parameter 1 of Foo.method does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testEnum1() throws Exception {
testTypes("/**@enum*/var a={BB:1,CC:2};\n" +
"/**@type {a}*/var d;d=a.BB;");
}
public void testEnum2() throws Exception {
testTypes("/**@enum*/var a={b:1}",
"enum key b must be a syntactic constant");
}
public void testEnum3() throws Exception {
testTypes("/**@enum*/var a={BB:1,BB:2}",
"variable a.BB redefined with type a.<number>, " +
"original definition at [testcode]:1 with type a.<number>");
}
public void testEnum4() throws Exception {
testTypes("/**@enum*/var a={BB:'string'}",
"assignment to property BB of enum{a}\n" +
"found : string\n" +
"required: number");
}
public void testEnum5() throws Exception {
testTypes("/**@enum {String}*/var a={BB:'string'}",
"assignment to property BB of enum{a}\n" +
"found : string\n" +
"required: (String|null)");
}
public void testEnum6() throws Exception {
testTypes("/**@enum*/var a={BB:1,CC:2};\n/**@type {!Array}*/var d;d=a.BB;",
"assignment\n" +
"found : a.<number>\n" +
"required: Array");
}
public void testEnum7() throws Exception {
testTypes("/** @enum */var a={AA:1,BB:2,CC:3};" +
"/** @type a */var b=a.D;",
"element D does not exist on this enum");
}
public void testEnum8() throws Exception {
testClosureTypesMultipleWarnings("/** @enum */var a=8;",
Lists.newArrayList(
"enum initializer must be an object literal or an enum",
"initializing variable\n" +
"found : number\n" +
"required: enum{a}"));
}
public void testEnum9() throws Exception {
testClosureTypesMultipleWarnings(
"var goog = {};" +
"/** @enum */goog.a=8;",
Lists.newArrayList(
"assignment to property a of goog\n" +
"found : number\n" +
"required: enum{goog.a}",
"enum initializer must be an object literal or an enum"));
}
public void testEnum10() throws Exception {
testTypes(
"/** @enum {number} */" +
"goog.K = { A : 3 };");
}
public void testEnum11() throws Exception {
testTypes(
"/** @enum {number} */" +
"goog.K = { 502 : 3 };");
}
public void testEnum12() throws Exception {
testTypes(
"/** @enum {number} */ var a = {};" +
"/** @enum */ var b = a;");
}
public void testEnum13() throws Exception {
testTypes(
"/** @enum {number} */ var a = {};" +
"/** @enum {string} */ var b = a;",
"incompatible enum element types\n" +
"found : number\n" +
"required: string");
}
public void testEnum14() throws Exception {
testTypes(
"/** @enum {number} */ var a = {FOO:5};" +
"/** @enum */ var b = a;" +
"var c = b.FOO;");
}
public void testEnum15() throws Exception {
testTypes(
"/** @enum {number} */ var a = {FOO:5};" +
"/** @enum */ var b = a;" +
"var c = b.BAR;",
"element BAR does not exist on this enum");
}
public void testEnum16() throws Exception {
testTypes("var goog = {};" +
"/**@enum*/goog .a={BB:1,BB:2}",
"variable goog.a.BB redefined with type goog.a.<number>, " +
"original definition at [testcode]:1 with type goog.a.<number>");
}
public void testEnum17() throws Exception {
testTypes("var goog = {};" +
"/**@enum*/goog.a={BB:'string'}",
"assignment to property BB of enum{goog.a}\n" +
"found : string\n" +
"required: number");
}
public void testEnum18() throws Exception {
testTypes("/**@enum*/ var E = {A: 1, B: 2};" +
"/** @param {!E} x\n@return {number} */\n" +
"var f = function(x) { return x; };");
}
public void testEnum19() throws Exception {
testTypes("/**@enum*/ var E = {A: 1, B: 2};" +
"/** @param {number} x\n@return {!E} */\n" +
"var f = function(x) { return x; };",
"inconsistent return type\n" +
"found : number\n" +
"required: E.<number>");
}
public void testEnum20() throws Exception {
testTypes("/**@enum*/ var E = {A: 1, B: 2}; var x = []; x[E.A] = 0;");
}
public void testEnum21() throws Exception {
Node n = parseAndTypeCheck(
"/** @enum {string} */ var E = {A : 'a', B : 'b'};\n" +
"/** @param {!E} x\n@return {!E} */ function f(x) { return x; }");
Node nodeX = n.getLastChild().getLastChild().getLastChild().getLastChild();
JSType typeE = nodeX.getJSType();
assertFalse(typeE.isObject());
assertFalse(typeE.isNullable());
}
public void testEnum22() throws Exception {
testTypes("/**@enum*/ var E = {A: 1, B: 2};" +
"/** @param {E} x \n* @return {number} */ function f(x) {return x}");
}
public void testEnum23() throws Exception {
testTypes("/**@enum*/ var E = {A: 1, B: 2};" +
"/** @param {E} x \n* @return {string} */ function f(x) {return x}",
"inconsistent return type\n" +
"found : E.<number>\n" +
"required: string");
}
public void testEnum24() throws Exception {
testTypes("/**@enum {Object} */ var E = {A: {}};" +
"/** @param {E} x \n* @return {!Object} */ function f(x) {return x}",
"inconsistent return type\n" +
"found : E.<(Object|null)>\n" +
"required: Object");
}
public void testEnum25() throws Exception {
testTypes("/**@enum {!Object} */ var E = {A: {}};" +
"/** @param {E} x \n* @return {!Object} */ function f(x) {return x}");
}
public void testEnum26() throws Exception {
testTypes("var a = {}; /**@enum*/ a.B = {A: 1, B: 2};" +
"/** @param {a.B} x \n* @return {number} */ function f(x) {return x}");
}
public void testEnum27() throws Exception {
// x is unknown
testTypes("/** @enum */ var A = {B: 1, C: 2}; " +
"function f(x) { return A == x; }");
}
public void testEnum28() throws Exception {
// x is unknown
testTypes("/** @enum */ var A = {B: 1, C: 2}; " +
"function f(x) { return A.B == x; }");
}
public void testEnum29() throws Exception {
testTypes("/** @enum */ var A = {B: 1, C: 2}; " +
"/** @return {number} */ function f() { return A; }",
"inconsistent return type\n" +
"found : enum{A}\n" +
"required: number");
}
public void testEnum30() throws Exception {
testTypes("/** @enum */ var A = {B: 1, C: 2}; " +
"/** @return {number} */ function f() { return A.B; }");
}
public void testEnum31() throws Exception {
testTypes("/** @enum */ var A = {B: 1, C: 2}; " +
"/** @return {A} */ function f() { return A; }",
"inconsistent return type\n" +
"found : enum{A}\n" +
"required: A.<number>");
}
public void testEnum32() throws Exception {
testTypes("/** @enum */ var A = {B: 1, C: 2}; " +
"/** @return {A} */ function f() { return A.B; }");
}
public void testEnum34() throws Exception {
testTypes("/** @enum */ var A = {B: 1, C: 2}; " +
"/** @param {number} x */ function f(x) { return x == A.B; }");
}
public void testEnum35() throws Exception {
testTypes("var a = a || {}; /** @enum */ a.b = {C: 1, D: 2};" +
"/** @return {a.b} */ function f() { return a.b.C; }");
}
public void testEnum36() throws Exception {
testTypes("var a = a || {}; /** @enum */ a.b = {C: 1, D: 2};" +
"/** @return {!a.b} */ function f() { return 1; }",
"inconsistent return type\n" +
"found : number\n" +
"required: a.b.<number>");
}
public void testEnum37() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @enum {number} */ goog.a = {};" +
"/** @enum */ var b = goog.a;");
}
public void testEnum38() throws Exception {
testTypes(
"/** @enum {MyEnum} */ var MyEnum = {};" +
"/** @param {MyEnum} x */ function f(x) {}",
"Parse error. Cycle detected in inheritance chain " +
"of type MyEnum");
}
public void testEnum39() throws Exception {
testTypes(
"/** @enum {Number} */ var MyEnum = {FOO: new Number(1)};" +
"/** @param {MyEnum} x \n * @return {number} */" +
"function f(x) { return x == MyEnum.FOO && MyEnum.FOO == x; }",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testEnum40() throws Exception {
testTypes(
"/** @enum {Number} */ var MyEnum = {FOO: new Number(1)};" +
"/** @param {number} x \n * @return {number} */" +
"function f(x) { return x == MyEnum.FOO && MyEnum.FOO == x; }",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testEnum41() throws Exception {
testTypes(
"/** @enum {number} */ var MyEnum = {/** @const */ FOO: 1};" +
"/** @return {string} */" +
"function f() { return MyEnum.FOO; }",
"inconsistent return type\n" +
"found : MyEnum.<number>\n" +
"required: string");
}
public void testEnum42() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"/** @enum {Object} */ var MyEnum = {FOO: {newProperty: 1, b: 2}};" +
"f(MyEnum.FOO.newProperty);");
}
public void testAliasedEnum1() throws Exception {
testTypes(
"/** @enum */ var YourEnum = {FOO: 3};" +
"/** @enum */ var MyEnum = YourEnum;" +
"/** @param {MyEnum} x */ function f(x) {} f(MyEnum.FOO);");
}
public void testAliasedEnum2() throws Exception {
testTypes(
"/** @enum */ var YourEnum = {FOO: 3};" +
"/** @enum */ var MyEnum = YourEnum;" +
"/** @param {YourEnum} x */ function f(x) {} f(MyEnum.FOO);");
}
public void testAliasedEnum3() throws Exception {
testTypes(
"/** @enum */ var YourEnum = {FOO: 3};" +
"/** @enum */ var MyEnum = YourEnum;" +
"/** @param {MyEnum} x */ function f(x) {} f(YourEnum.FOO);");
}
public void testAliasedEnum4() throws Exception {
testTypes(
"/** @enum */ var YourEnum = {FOO: 3};" +
"/** @enum */ var MyEnum = YourEnum;" +
"/** @param {YourEnum} x */ function f(x) {} f(YourEnum.FOO);");
}
public void testAliasedEnum5() throws Exception {
testTypes(
"/** @enum */ var YourEnum = {FOO: 3};" +
"/** @enum */ var MyEnum = YourEnum;" +
"/** @param {string} x */ function f(x) {} f(MyEnum.FOO);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : YourEnum.<number>\n" +
"required: string");
}
public void testBackwardsEnumUse1() throws Exception {
testTypes(
"/** @return {string} */ function f() { return MyEnum.FOO; }" +
"/** @enum {string} */ var MyEnum = {FOO: 'x'};");
}
public void testBackwardsEnumUse2() throws Exception {
testTypes(
"/** @return {number} */ function f() { return MyEnum.FOO; }" +
"/** @enum {string} */ var MyEnum = {FOO: 'x'};",
"inconsistent return type\n" +
"found : MyEnum.<string>\n" +
"required: number");
}
public void testBackwardsEnumUse3() throws Exception {
testTypes(
"/** @return {string} */ function f() { return MyEnum.FOO; }" +
"/** @enum {string} */ var YourEnum = {FOO: 'x'};" +
"/** @enum {string} */ var MyEnum = YourEnum;");
}
public void testBackwardsEnumUse4() throws Exception {
testTypes(
"/** @return {number} */ function f() { return MyEnum.FOO; }" +
"/** @enum {string} */ var YourEnum = {FOO: 'x'};" +
"/** @enum {string} */ var MyEnum = YourEnum;",
"inconsistent return type\n" +
"found : YourEnum.<string>\n" +
"required: number");
}
public void testBackwardsEnumUse5() throws Exception {
testTypes(
"/** @return {string} */ function f() { return MyEnum.BAR; }" +
"/** @enum {string} */ var YourEnum = {FOO: 'x'};" +
"/** @enum {string} */ var MyEnum = YourEnum;",
"element BAR does not exist on this enum");
}
public void testBackwardsTypedefUse1() throws Exception {
testTypes(
"/** @this {MyTypedef} */ function f() {}" +
"/** @typedef {string} */ var MyTypedef;",
"@this type of a function must be an object\n" +
"Actual type: string");
}
public void testBackwardsTypedefUse2() throws Exception {
testTypes(
"/** @this {MyTypedef} */ function f() {}" +
"/** @typedef {!(Date|Array)} */ var MyTypedef;");
}
public void testBackwardsTypedefUse3() throws Exception {
testTypes(
"/** @this {MyTypedef} */ function f() {}" +
"/** @typedef {(Date|string)} */ var MyTypedef;",
"@this type of a function must be an object\n" +
"Actual type: (Date|null|string)");
}
public void testBackwardsTypedefUse4() throws Exception {
testTypes(
"/** @return {MyTypedef} */ function f() { return null; }" +
"/** @typedef {string} */ var MyTypedef;",
"inconsistent return type\n" +
"found : null\n" +
"required: string");
}
public void testBackwardsTypedefUse6() throws Exception {
testTypes(
"/** @return {goog.MyTypedef} */ function f() { return null; }" +
"var goog = {};" +
"/** @typedef {string} */ goog.MyTypedef;",
"inconsistent return type\n" +
"found : null\n" +
"required: string");
}
public void testBackwardsTypedefUse7() throws Exception {
testTypes(
"/** @return {goog.MyTypedef} */ function f() { return null; }" +
"var goog = {};" +
"/** @typedef {Object} */ goog.MyTypedef;");
}
public void testBackwardsTypedefUse8() throws Exception {
// Tehnically, this isn't quite right, because the JS runtime
// will coerce null -> the global object. But we'll punt on that for now.
testTypes(
"/** @param {!Array} x */ function g(x) {}" +
"/** @this {goog.MyTypedef} */ function f() { g(this); }" +
"var goog = {};" +
"/** @typedef {(Array|null|undefined)} */ goog.MyTypedef;");
}
public void testBackwardsTypedefUse9() throws Exception {
testTypes(
"/** @param {!Array} x */ function g(x) {}" +
"/** @this {goog.MyTypedef} */ function f() { g(this); }" +
"var goog = {};" +
"/** @typedef {(Error|null|undefined)} */ goog.MyTypedef;",
"actual parameter 1 of g does not match formal parameter\n" +
"found : Error\n" +
"required: Array");
}
public void testBackwardsTypedefUse10() throws Exception {
testTypes(
"/** @param {goog.MyEnum} x */ function g(x) {}" +
"var goog = {};" +
"/** @enum {goog.MyTypedef} */ goog.MyEnum = {FOO: 1};" +
"/** @typedef {number} */ goog.MyTypedef;" +
"g(1);",
"actual parameter 1 of g does not match formal parameter\n" +
"found : number\n" +
"required: goog.MyEnum.<number>");
}
public void testBackwardsConstructor1() throws Exception {
testTypes(
"function f() { (new Foo(true)); }" +
"/** \n * @constructor \n * @param {number} x */" +
"var Foo = function(x) {};",
"actual parameter 1 of Foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testBackwardsConstructor2() throws Exception {
testTypes(
"function f() { (new Foo(true)); }" +
"/** \n * @constructor \n * @param {number} x */" +
"var YourFoo = function(x) {};" +
"/** \n * @constructor \n * @param {number} x */" +
"var Foo = YourFoo;",
"actual parameter 1 of Foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testMinimalConstructorAnnotation() throws Exception {
testTypes("/** @constructor */function Foo(){}");
}
public void testGoodExtends1() throws Exception {
// A minimal @extends example
testTypes("/** @constructor */function base() {}\n" +
"/** @constructor\n * @extends {base} */function derived() {}\n");
}
public void testGoodExtends2() throws Exception {
testTypes("/** @constructor\n * @extends base */function derived() {}\n" +
"/** @constructor */function base() {}\n");
}
public void testGoodExtends3() throws Exception {
testTypes("/** @constructor\n * @extends {Object} */function base() {}\n" +
"/** @constructor\n * @extends {base} */function derived() {}\n");
}
public void testGoodExtends4() throws Exception {
// Ensure that @extends actually sets the base type of a constructor
// correctly. Because this isn't part of the human-readable Function
// definition, we need to crawl the prototype chain (eww).
Node n = parseAndTypeCheck(
"var goog = {};\n" +
"/** @constructor */goog.Base = function(){};\n" +
"/** @constructor\n" +
" * @extends {goog.Base} */goog.Derived = function(){};\n");
Node subTypeName = n.getLastChild().getLastChild().getFirstChild();
assertEquals("goog.Derived", subTypeName.getQualifiedName());
FunctionType subCtorType =
(FunctionType) subTypeName.getNext().getJSType();
assertEquals("goog.Derived", subCtorType.getInstanceType().toString());
JSType superType = subCtorType.getPrototype().getImplicitPrototype();
assertEquals("goog.Base", superType.toString());
}
public void testGoodExtends5() throws Exception {
// we allow for the extends annotation to be placed first
testTypes("/** @constructor */function base() {}\n" +
"/** @extends {base}\n * @constructor */function derived() {}\n");
}
public void testGoodExtends6() throws Exception {
testFunctionType(
CLOSURE_DEFS +
"/** @constructor */function base() {}\n" +
"/** @return {number} */ " +
" base.prototype.foo = function() { return 1; };\n" +
"/** @extends {base}\n * @constructor */function derived() {}\n" +
"goog.inherits(derived, base);",
"derived.superClass_.foo",
"function (this:base): number");
}
public void testGoodExtends7() throws Exception {
testFunctionType(
"Function.prototype.inherits = function(x) {};" +
"/** @constructor */function base() {}\n" +
"/** @extends {base}\n * @constructor */function derived() {}\n" +
"derived.inherits(base);",
"(new derived).constructor",
"function (new:derived): undefined");
}
public void testGoodExtends8() throws Exception {
testTypes("/** @constructor \n @extends {Base} */ function Sub() {}" +
"/** @return {number} */ function f() { return (new Sub()).foo; }" +
"/** @constructor */ function Base() {}" +
"/** @type {boolean} */ Base.prototype.foo = true;",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testGoodExtends9() throws Exception {
testTypes(
"/** @constructor */ function Super() {}" +
"Super.prototype.foo = function() {};" +
"/** @constructor \n * @extends {Super} */ function Sub() {}" +
"Sub.prototype = new Super();" +
"/** @override */ Sub.prototype.foo = function() {};");
}
public void testGoodExtends10() throws Exception {
testTypes(
"/** @constructor */ function Super() {}" +
"/** @constructor \n * @extends {Super} */ function Sub() {}" +
"Sub.prototype = new Super();" +
"/** @return {Super} */ function foo() { return new Sub(); }");
}
public void testGoodExtends11() throws Exception {
testTypes(
"/** @constructor */ function Super() {}" +
"/** @param {boolean} x */ Super.prototype.foo = function(x) {};" +
"/** @constructor \n * @extends {Super} */ function Sub() {}" +
"Sub.prototype = new Super();" +
"(new Sub()).foo(0);",
"actual parameter 1 of Super.prototype.foo " +
"does not match formal parameter\n" +
"found : number\n" +
"required: boolean");
}
public void testGoodExtends12() throws Exception {
testTypes(
"/** @constructor \n * @extends {Super} */ function Sub() {}" +
"/** @constructor \n * @extends {Sub} */ function Sub2() {}" +
"/** @constructor */ function Super() {}" +
"/** @param {Super} x */ function foo(x) {}" +
"foo(new Sub2());");
}
public void testGoodExtends13() throws Exception {
testTypes(
"/** @constructor \n * @extends {B} */ function C() {}" +
"/** @constructor \n * @extends {D} */ function E() {}" +
"/** @constructor \n * @extends {C} */ function D() {}" +
"/** @constructor \n * @extends {A} */ function B() {}" +
"/** @constructor */ function A() {}" +
"/** @param {number} x */ function f(x) {} f(new E());",
"actual parameter 1 of f does not match formal parameter\n" +
"found : E\n" +
"required: number");
}
public void testGoodExtends14() throws Exception {
testTypes(
CLOSURE_DEFS +
"/** @param {Function} f */ function g(f) {" +
" /** @constructor */ function NewType() {};" +
" goog.inherits(NewType, f);" +
" (new NewType());" +
"}");
}
public void testGoodExtends15() throws Exception {
testTypes(
CLOSURE_DEFS +
"/** @constructor */ function OldType() {}" +
"/** @param {?function(new:OldType)} f */ function g(f) {" +
" /**\n" +
" * @constructor\n" +
" * @extends {OldType}\n" +
" */\n" +
" function NewType() {};" +
" goog.inherits(NewType, f);" +
" NewType.prototype.method = function() {" +
" NewType.superClass_.foo.call(this);" +
" };" +
"}",
"Property foo never defined on OldType.prototype");
}
public void testGoodExtends16() throws Exception {
testTypes(
CLOSURE_DEFS +
"/** @param {Function} f */ function g(f) {" +
" /** @constructor */ function NewType() {};" +
" goog.inherits(f, NewType);" +
" (new NewType());" +
"}");
}
public void testBadExtends1() throws Exception {
testTypes("/** @constructor */function base() {}\n" +
"/** @constructor\n * @extends {not_base} */function derived() {}\n",
"Bad type annotation. Unknown type not_base");
}
public void testBadExtends2() throws Exception {
testTypes("/** @constructor */function base() {\n" +
"/** @type {!Number}*/\n" +
"this.baseMember = new Number(4);\n" +
"}\n" +
"/** @constructor\n" +
" * @extends {base} */function derived() {}\n" +
"/** @param {!String} x*/\n" +
"function foo(x){ }\n" +
"/** @type {!derived}*/var y;\n" +
"foo(y.baseMember);\n",
"actual parameter 1 of foo does not match formal parameter\n" +
"found : Number\n" +
"required: String");
}
public void testBadExtends3() throws Exception {
testTypes("/** @extends {Object} */function base() {}",
"@extends used without @constructor or @interface for base");
}
public void testBadExtends4() throws Exception {
// If there's a subclass of a class with a bad extends,
// we only want to warn about the first one.
testTypes(
"/** @constructor \n * @extends {bad} */ function Sub() {}" +
"/** @constructor \n * @extends {Sub} */ function Sub2() {}" +
"/** @param {Sub} x */ function foo(x) {}" +
"foo(new Sub2());",
"Bad type annotation. Unknown type bad");
}
public void testLateExtends() throws Exception {
testTypes(
CLOSURE_DEFS +
"/** @constructor */ function Foo() {}\n" +
"Foo.prototype.foo = function() {};\n" +
"/** @constructor */function Bar() {}\n" +
"goog.inherits(Foo, Bar);\n",
"Missing @extends tag on type Foo");
}
public void testSuperclassMatch() throws Exception {
compiler.getOptions().setCodingConvention(new GoogleCodingConvention());
testTypes("/** @constructor */ var Foo = function() {};\n" +
"/** @constructor \n @extends Foo */ var Bar = function() {};\n" +
"Bar.inherits = function(x){};" +
"Bar.inherits(Foo);\n");
}
public void testSuperclassMatchWithMixin() throws Exception {
compiler.getOptions().setCodingConvention(new GoogleCodingConvention());
testTypes("/** @constructor */ var Foo = function() {};\n" +
"/** @constructor */ var Baz = function() {};\n" +
"/** @constructor \n @extends Foo */ var Bar = function() {};\n" +
"Bar.inherits = function(x){};" +
"Bar.mixin = function(y){};" +
"Bar.inherits(Foo);\n" +
"Bar.mixin(Baz);\n");
}
public void testSuperclassMismatch1() throws Exception {
compiler.getOptions().setCodingConvention(new GoogleCodingConvention());
testTypes("/** @constructor */ var Foo = function() {};\n" +
"/** @constructor \n @extends Object */ var Bar = function() {};\n" +
"Bar.inherits = function(x){};" +
"Bar.inherits(Foo);\n",
"Missing @extends tag on type Bar");
}
public void testSuperclassMismatch2() throws Exception {
compiler.getOptions().setCodingConvention(new GoogleCodingConvention());
testTypes("/** @constructor */ var Foo = function(){};\n" +
"/** @constructor */ var Bar = function(){};\n" +
"Bar.inherits = function(x){};" +
"Bar.inherits(Foo);",
"Missing @extends tag on type Bar");
}
public void testSuperClassDefinedAfterSubClass1() throws Exception {
testTypes(
"/** @constructor \n * @extends {Base} */ function A() {}" +
"/** @constructor \n * @extends {Base} */ function B() {}" +
"/** @constructor */ function Base() {}" +
"/** @param {A|B} x \n * @return {B|A} */ " +
"function foo(x) { return x; }");
}
public void testSuperClassDefinedAfterSubClass2() throws Exception {
testTypes(
"/** @constructor \n * @extends {Base} */ function A() {}" +
"/** @constructor \n * @extends {Base} */ function B() {}" +
"/** @param {A|B} x \n * @return {B|A} */ " +
"function foo(x) { return x; }" +
"/** @constructor */ function Base() {}");
}
public void testDirectPrototypeAssignment1() throws Exception {
testTypes(
"/** @constructor */ function Base() {}" +
"Base.prototype.foo = 3;" +
"/** @constructor \n * @extends {Base} */ function A() {}" +
"A.prototype = new Base();" +
"/** @return {string} */ function foo() { return (new A).foo; }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testDirectPrototypeAssignment2() throws Exception {
// This ensures that we don't attach property 'foo' onto the Base
// instance object.
testTypes(
"/** @constructor */ function Base() {}" +
"/** @constructor \n * @extends {Base} */ function A() {}" +
"A.prototype = new Base();" +
"A.prototype.foo = 3;" +
"/** @return {string} */ function foo() { return (new Base).foo; }");
}
public void testDirectPrototypeAssignment3() throws Exception {
// This verifies that the compiler doesn't crash if the user
// overwrites the prototype of a global variable in a local scope.
testTypes(
"/** @constructor */ var MainWidgetCreator = function() {};" +
"/** @param {Function} ctor */" +
"function createMainWidget(ctor) {" +
" /** @constructor */ function tempCtor() {};" +
" tempCtor.prototype = ctor.prototype;" +
" MainWidgetCreator.superClass_ = ctor.prototype;" +
" MainWidgetCreator.prototype = new tempCtor();" +
"}");
}
public void testGoodImplements1() throws Exception {
testTypes("/** @interface */function Disposable() {}\n" +
"/** @implements {Disposable}\n * @constructor */function f() {}");
}
public void testGoodImplements2() throws Exception {
testTypes("/** @interface */function Base1() {}\n" +
"/** @interface */function Base2() {}\n" +
"/** @constructor\n" +
" * @implements {Base1}\n" +
" * @implements {Base2}\n" +
" */ function derived() {}");
}
public void testGoodImplements3() throws Exception {
testTypes("/** @interface */function Disposable() {}\n" +
"/** @constructor \n @implements {Disposable} */function f() {}");
}
public void testGoodImplements4() throws Exception {
testTypes("var goog = {};" +
"/** @type {!Function} */" +
"goog.abstractMethod = function() {};" +
"/** @interface */\n" +
"goog.Disposable = goog.abstractMethod;" +
"goog.Disposable.prototype.dispose = goog.abstractMethod;" +
"/** @implements {goog.Disposable}\n * @constructor */" +
"goog.SubDisposable = function() {};" +
"/** @inheritDoc */ " +
"goog.SubDisposable.prototype.dispose = function() {};");
}
public void testGoodImplements5() throws Exception {
testTypes(
"/** @interface */\n" +
"goog.Disposable = function() {};" +
"/** @type {Function} */" +
"goog.Disposable.prototype.dispose = function() {};" +
"/** @implements {goog.Disposable}\n * @constructor */" +
"goog.SubDisposable = function() {};" +
"/** @param {number} key \n @override */ " +
"goog.SubDisposable.prototype.dispose = function(key) {};");
}
public void testGoodImplements6() throws Exception {
testTypes(
"var myNullFunction = function() {};" +
"/** @interface */\n" +
"goog.Disposable = function() {};" +
"/** @return {number} */" +
"goog.Disposable.prototype.dispose = myNullFunction;" +
"/** @implements {goog.Disposable}\n * @constructor */" +
"goog.SubDisposable = function() {};" +
"/** @return {number} \n @override */ " +
"goog.SubDisposable.prototype.dispose = function() { return 0; };");
}
public void testGoodImplements7() throws Exception {
testTypes(
"var myNullFunction = function() {};" +
"/** @interface */\n" +
"goog.Disposable = function() {};" +
"/** @return {number} */" +
"goog.Disposable.prototype.dispose = function() {};" +
"/** @implements {goog.Disposable}\n * @constructor */" +
"goog.SubDisposable = function() {};" +
"/** @return {number} \n @override */ " +
"goog.SubDisposable.prototype.dispose = function() { return 0; };");
}
public void testBadImplements1() throws Exception {
testTypes("/** @interface */function Base1() {}\n" +
"/** @interface */function Base2() {}\n" +
"/** @constructor\n" +
" * @implements {nonExistent}\n" +
" * @implements {Base2}\n" +
" */ function derived() {}",
"Bad type annotation. Unknown type nonExistent");
}
public void testBadImplements2() throws Exception {
testTypes("/** @interface */function Disposable() {}\n" +
"/** @implements {Disposable}\n */function f() {}",
"@implements used without @constructor or @interface for f");
}
public void testBadImplements3() throws Exception {
testTypes(
"var goog = {};" +
"/** @type {!Function} */ goog.abstractMethod = function(){};" +
"/** @interface */ var Disposable = goog.abstractMethod;" +
"Disposable.prototype.method = goog.abstractMethod;" +
"/** @implements {Disposable}\n * @constructor */function f() {}",
"property method on interface Disposable is not implemented by type f");
}
public void testBadImplements4() throws Exception {
testTypes("/** @interface */function Disposable() {}\n" +
"/** @implements {Disposable}\n * @interface */function f() {}",
"f cannot implement this type; an interface can only extend, " +
"but not implement interfaces");
}
public void testBadImplements5() throws Exception {
testTypes("/** @interface */function Disposable() {}\n" +
"/** @type {number} */ Disposable.prototype.bar = function() {};",
"assignment to property bar of Disposable.prototype\n" +
"found : function (): undefined\n" +
"required: number");
}
public void testBadImplements6() throws Exception {
testClosureTypesMultipleWarnings(
"/** @interface */function Disposable() {}\n" +
"/** @type {function()} */ Disposable.prototype.bar = 3;",
Lists.newArrayList(
"assignment to property bar of Disposable.prototype\n" +
"found : number\n" +
"required: function (): ?",
"interface members can only be empty property declarations, " +
"empty functions, or goog.abstractMethod"));
}
public void testInterfaceExtends() throws Exception {
testTypes("/** @interface */function A() {}\n" +
"/** @interface \n * @extends {A} */function B() {}\n" +
"/** @constructor\n" +
" * @implements {B}\n" +
" */ function derived() {}");
}
public void testBadInterfaceExtends1() throws Exception {
testTypes("/** @interface \n * @extends {nonExistent} */function A() {}",
"Bad type annotation. Unknown type nonExistent");
}
public void testBadInterfaceExtends2() throws Exception {
testTypes("/** @constructor */function A() {}\n" +
"/** @interface \n * @extends {A} */function B() {}",
"B cannot extend this type; a constructor can only extend objects " +
"and an interface can only extend interfaces");
}
public void testBadInterfaceExtends3() throws Exception {
testTypes("/** @interface */function A() {}\n" +
"/** @constructor \n * @extends {A} */function B() {}",
"B cannot extend this type; a constructor can only extend objects " +
"and an interface can only extend interfaces");
}
public void testBadInterfaceExtends4() throws Exception {
// TODO(user): This should be detected as an error. Even if we enforce
// that A cannot be used in the assignment, we should still detect the
// inheritance chain as invalid.
testTypes("/** @interface */function A() {}\n" +
"/** @constructor */function B() {}\n" +
"B.prototype = A;");
}
public void testBadInterfaceExtends5() throws Exception {
// TODO(user): This should be detected as an error. Even if we enforce
// that A cannot be used in the assignment, we should still detect the
// inheritance chain as invalid.
testTypes("/** @constructor */function A() {}\n" +
"/** @interface */function B() {}\n" +
"B.prototype = A;");
}
public void testBadImplementsAConstructor() throws Exception {
testTypes("/** @constructor */function A() {}\n" +
"/** @constructor \n * @implements {A} */function B() {}",
"can only implement interfaces");
}
public void testBadImplementsNonInterfaceType() throws Exception {
testTypes("/** @constructor \n * @implements {Boolean} */function B() {}",
"can only implement interfaces");
}
public void testBadImplementsNonObjectType() throws Exception {
testTypes("/** @constructor \n * @implements {string} */function S() {}",
"can only implement interfaces");
}
public void testInterfaceAssignment1() throws Exception {
testTypes("/** @interface */var I = function() {};\n" +
"/** @constructor\n@implements {I} */var T = function() {};\n" +
"var t = new T();\n" +
"/** @type {!I} */var i = t;");
}
public void testInterfaceAssignment2() throws Exception {
testTypes("/** @interface */var I = function() {};\n" +
"/** @constructor */var T = function() {};\n" +
"var t = new T();\n" +
"/** @type {!I} */var i = t;",
"initializing variable\n" +
"found : T\n" +
"required: I");
}
public void testInterfaceAssignment3() throws Exception {
testTypes("/** @interface */var I = function() {};\n" +
"/** @constructor\n@implements {I} */var T = function() {};\n" +
"var t = new T();\n" +
"/** @type {I|number} */var i = t;");
}
public void testInterfaceAssignment4() throws Exception {
testTypes("/** @interface */var I1 = function() {};\n" +
"/** @interface */var I2 = function() {};\n" +
"/** @constructor\n@implements {I1} */var T = function() {};\n" +
"var t = new T();\n" +
"/** @type {I1|I2} */var i = t;");
}
public void testInterfaceAssignment5() throws Exception {
testTypes("/** @interface */var I1 = function() {};\n" +
"/** @interface */var I2 = function() {};\n" +
"/** @constructor\n@implements {I1}\n@implements {I2}*/" +
"var T = function() {};\n" +
"var t = new T();\n" +
"/** @type {I1} */var i1 = t;\n" +
"/** @type {I2} */var i2 = t;\n");
}
public void testInterfaceAssignment6() throws Exception {
testTypes("/** @interface */var I1 = function() {};\n" +
"/** @interface */var I2 = function() {};\n" +
"/** @constructor\n@implements {I1} */var T = function() {};\n" +
"/** @type {!I1} */var i1 = new T();\n" +
"/** @type {!I2} */var i2 = i1;\n",
"initializing variable\n" +
"found : I1\n" +
"required: I2");
}
public void testInterfaceAssignment7() throws Exception {
testTypes("/** @interface */var I1 = function() {};\n" +
"/** @interface\n@extends {I1}*/var I2 = function() {};\n" +
"/** @constructor\n@implements {I2}*/var T = function() {};\n" +
"var t = new T();\n" +
"/** @type {I1} */var i1 = t;\n" +
"/** @type {I2} */var i2 = t;\n" +
"i1 = i2;\n");
}
public void testInterfaceAssignment8() throws Exception {
testTypes("/** @interface */var I = function() {};\n" +
"/** @type {I} */var i;\n" +
"/** @type {Object} */var o = i;\n" +
"new Object().prototype = i.prototype;");
}
public void testInterfaceAssignment9() throws Exception {
testTypes("/** @interface */var I = function() {};\n" +
"/** @return {I?} */function f() { return null; }\n" +
"/** @type {!I} */var i = f();\n",
"initializing variable\n" +
"found : (I|null)\n" +
"required: I");
}
public void testInterfaceAssignment10() throws Exception {
testTypes("/** @interface */var I1 = function() {};\n" +
"/** @interface */var I2 = function() {};\n" +
"/** @constructor\n@implements {I2} */var T = function() {};\n" +
"/** @return {!I1|!I2} */function f() { return new T(); }\n" +
"/** @type {!I1} */var i1 = f();\n",
"initializing variable\n" +
"found : (I1|I2)\n" +
"required: I1");
}
public void testInterfaceAssignment11() throws Exception {
testTypes("/** @interface */var I1 = function() {};\n" +
"/** @interface */var I2 = function() {};\n" +
"/** @constructor */var T = function() {};\n" +
"/** @return {!I1|!I2|!T} */function f() { return new T(); }\n" +
"/** @type {!I1} */var i1 = f();\n",
"initializing variable\n" +
"found : (I1|I2|T)\n" +
"required: I1");
}
public void testInterfaceAssignment12() throws Exception {
testTypes("/** @interface */var I = function() {};\n" +
"/** @constructor\n@implements{I}*/var T1 = function() {};\n" +
"/** @constructor\n@extends {T1}*/var T2 = function() {};\n" +
"/** @return {I} */function f() { return new T2(); }");
}
public void testInterfaceAssignment13() throws Exception {
testTypes("/** @interface */var I = function() {};\n" +
"/** @constructor\n@implements {I}*/var T = function() {};\n" +
"/** @constructor */function Super() {};\n" +
"/** @return {I} */Super.prototype.foo = " +
"function() { return new T(); };\n" +
"/** @constructor\n@extends {Super} */function Sub() {}\n" +
"/** @override\n@return {T} */Sub.prototype.foo = " +
"function() { return new T(); };\n");
}
public void testGetprop1() throws Exception {
testTypes("/** @return {void}*/function foo(){foo().bar;}",
"No properties on this expression\n" +
"found : undefined\n" +
"required: Object");
}
public void testGetprop2() throws Exception {
testTypes("var x = null; x.alert();",
"No properties on this expression\n" +
"found : null\n" +
"required: Object");
}
public void testGetprop3() throws Exception {
testTypes(
"/** @constructor */ " +
"function Foo() { /** @type {?Object} */ this.x = null; }" +
"Foo.prototype.initX = function() { this.x = {foo: 1}; };" +
"Foo.prototype.bar = function() {" +
" if (this.x == null) { this.initX(); alert(this.x.foo); }" +
"};");
}
public void testArrayAccess1() throws Exception {
testTypes("var a = []; var b = a['hi'];");
}
public void testArrayAccess2() throws Exception {
testTypes("var a = []; var b = a[[1,2]];",
"array access\n" +
"found : Array\n" +
"required: number");
}
public void testArrayAccess3() throws Exception {
testTypes("var bar = [];" +
"/** @return {void} */function baz(){};" +
"var foo = bar[baz()];",
"array access\n" +
"found : undefined\n" +
"required: number");
}
public void testArrayAccess4() throws Exception {
testTypes("/**@return {!Array}*/function foo(){};var bar = foo()[foo()];",
"array access\n" +
"found : Array\n" +
"required: number");
}
public void testArrayAccess6() throws Exception {
testTypes("var bar = null[1];",
"only arrays or objects can be accessed\n" +
"found : null\n" +
"required: Object");
}
public void testArrayAccess7() throws Exception {
testTypes("var bar = void 0; bar[0];",
"only arrays or objects can be accessed\n" +
"found : undefined\n" +
"required: Object");
}
public void testArrayAccess8() throws Exception {
// Verifies that we don't emit two warnings, because
// the var has been dereferenced after the first one.
testTypes("var bar = void 0; bar[0]; bar[1];",
"only arrays or objects can be accessed\n" +
"found : undefined\n" +
"required: Object");
}
public void testArrayAccess9() throws Exception {
testTypes("/** @return {?Array} */ function f() { return []; }" +
"f()[{}]",
"array access\n" +
"found : {}\n" +
"required: number");
}
public void testPropAccess() throws Exception {
testTypes("/** @param {*} x */var f = function(x) {\n" +
"var o = String(x);\n" +
"if (typeof o['a'] != 'undefined') { return o['a']; }\n" +
"return null;\n" +
"};");
}
public void testPropAccess2() throws Exception {
testTypes("var bar = void 0; bar.baz;",
"No properties on this expression\n" +
"found : undefined\n" +
"required: Object");
}
public void testPropAccess3() throws Exception {
// Verifies that we don't emit two warnings, because
// the var has been dereferenced after the first one.
testTypes("var bar = void 0; bar.baz; bar.bax;",
"No properties on this expression\n" +
"found : undefined\n" +
"required: Object");
}
public void testPropAccess4() throws Exception {
testTypes("/** @param {*} x */ function f(x) { return x['hi']; }");
}
public void testSwitchCase1() throws Exception {
testTypes("/**@type number*/var a;" +
"/**@type string*/var b;" +
"switch(a){case b:;}",
"case expression doesn't match switch\n" +
"found : string\n" +
"required: number");
}
public void testSwitchCase2() throws Exception {
testTypes("var a = null; switch (typeof a) { case 'foo': }");
}
public void testVar1() throws Exception {
TypeCheckResult p =
parseAndTypeCheckWithScope("/** @type {(string,null)} */var a = null");
assertEquals(createUnionType(STRING_TYPE, NULL_TYPE),
p.scope.getVar("a").getType());
}
public void testVar2() throws Exception {
testTypes("/** @type {Function} */ var a = function(){}");
}
public void testVar3() throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope("var a = 3;");
assertEquals(NUMBER_TYPE, p.scope.getVar("a").getType());
}
public void testVar4() throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope(
"var a = 3; a = 'string';");
assertEquals(createUnionType(STRING_TYPE, NUMBER_TYPE),
p.scope.getVar("a").getType());
}
public void testVar5() throws Exception {
testTypes("var goog = {};" +
"/** @type string */goog.foo = 'hello';" +
"/** @type number */var a = goog.foo;",
"initializing variable\n" +
"found : string\n" +
"required: number");
}
public void testVar6() throws Exception {
testTypes(
"function f() {" +
" return function() {" +
" /** @type {!Date} */" +
" var a = 7;" +
" };" +
"}",
"initializing variable\n" +
"found : number\n" +
"required: Date");
}
public void testVar7() throws Exception {
testTypes("/** @type number */var a, b;",
"declaration of multiple variables with shared type information");
}
public void testVar8() throws Exception {
testTypes("var a, b;");
}
public void testVar9() throws Exception {
testTypes("/** @enum */var a;",
"enum initializer must be an object literal or an enum");
}
public void testVar10() throws Exception {
testTypes("/** @type !Number */var foo = 'abc';",
"initializing variable\n" +
"found : string\n" +
"required: Number");
}
public void testVar11() throws Exception {
testTypes("var /** @type !Date */foo = 'abc';",
"initializing variable\n" +
"found : string\n" +
"required: Date");
}
public void testVar12() throws Exception {
testTypes("var /** @type !Date */foo = 'abc', " +
"/** @type !RegExp */bar = 5;",
new String[] {
"initializing variable\n" +
"found : string\n" +
"required: Date",
"initializing variable\n" +
"found : number\n" +
"required: RegExp"});
}
public void testVar13() throws Exception {
// this caused an NPE
testTypes("var /** @type number */a,a;");
}
public void testVar14() throws Exception {
testTypes("/** @return {number} */ function f() { var x; return x; }",
"inconsistent return type\n" +
"found : undefined\n" +
"required: number");
}
public void testVar15() throws Exception {
testTypes("/** @return {number} */" +
"function f() { var x = x || {}; return x; }",
"inconsistent return type\n" +
"found : {}\n" +
"required: number");
}
public void testAssign1() throws Exception {
testTypes("var goog = {};" +
"/** @type number */goog.foo = 'hello';",
"assignment to property foo of goog\n" +
"found : string\n" +
"required: number");
}
public void testAssign2() throws Exception {
testTypes("var goog = {};" +
"/** @type number */goog.foo = 3;" +
"goog.foo = 'hello';",
"assignment to property foo of goog\n" +
"found : string\n" +
"required: number");
}
public void testAssign3() throws Exception {
testTypes("var goog = {};" +
"/** @type number */goog.foo = 3;" +
"goog.foo = 4;");
}
public void testAssign4() throws Exception {
testTypes("var goog = {};" +
"goog.foo = 3;" +
"goog.foo = 'hello';");
}
public void testAssignInference() throws Exception {
testTypes(
"/**" +
" * @param {Array} x" +
" * @return {number}" +
" */" +
"function f(x) {" +
" var y = null;" +
" y = x[0];" +
" if (y == null) { return 4; } else { return 6; }" +
"}");
}
public void testOr1() throws Exception {
testTypes("/** @type number */var a;" +
"/** @type number */var b;" +
"a + b || undefined;");
}
public void testOr2() throws Exception {
testTypes("/** @type number */var a;" +
"/** @type number */var b;" +
"/** @type number */var c = a + b || undefined;",
"initializing variable\n" +
"found : (number|undefined)\n" +
"required: number");
}
public void testOr3() throws Exception {
testTypes("/** @type {(number, undefined)} */var a;" +
"/** @type number */var c = a || 3;");
}
/**
* Test that type inference continues with the right side,
* when no short-circuiting is possible.
* See bugid 1205387 for more details.
*/
public void testOr4() throws Exception {
testTypes("/**@type {number} */var x;x=null || \"a\";",
"assignment\n" +
"found : string\n" +
"required: number");
}
/**
* @see #testOr4()
*/
public void testOr5() throws Exception {
testTypes("/**@type {number} */var x;x=undefined || \"a\";",
"assignment\n" +
"found : string\n" +
"required: number");
}
public void testAnd1() throws Exception {
testTypes("/** @type number */var a;" +
"/** @type number */var b;" +
"a + b && undefined;");
}
public void testAnd2() throws Exception {
testTypes("/** @type number */var a;" +
"/** @type number */var b;" +
"/** @type number */var c = a + b && undefined;",
"initializing variable\n" +
"found : (number|undefined)\n" +
"required: number");
}
public void testAnd3() throws Exception {
testTypes("/** @type {(!Array, undefined)} */var a;" +
"/** @type number */var c = a && undefined;",
"initializing variable\n" +
"found : undefined\n" +
"required: number");
}
public void testAnd4() throws Exception {
testTypes("/** @param {number} x */function f(x){};\n" +
"/** @type null */var x; /** @type {number?} */var y;\n" +
"if (x && y) { f(y) }");
}
public void testAnd5() throws Exception {
testTypes("/** @param {number} x\n@param {string} y*/function f(x,y){};\n" +
"/** @type {number?} */var x; /** @type {string?} */var y;\n" +
"if (x && y) { f(x, y) }");
}
public void testAnd6() throws Exception {
testTypes("/** @param {number} x */function f(x){};\n" +
"/** @type {number|undefined} */var x;\n" +
"if (x && f(x)) { f(x) }");
}
public void testAnd7() throws Exception {
// TODO(user): a deterministic warning should be generated for this
// case since x && x is always false. The implementation of this requires
// a more precise handling of a null value within a variable's type.
// Currently, a null value defaults to ? which passes every check.
testTypes("/** @type null */var x; if (x && x) {}");
}
public void testHook() throws Exception {
testTypes("/**@return {void}*/function foo(){ var x=foo()?a:b; }");
}
public void testHookRestrictsType1() throws Exception {
testTypes("/** @return {(string,null)} */" +
"function f() { return null;}" +
"/** @type {(string,null)} */ var a = f();" +
"/** @type string */" +
"var b = a ? a : 'default';");
}
public void testHookRestrictsType2() throws Exception {
testTypes("/** @type {String} */" +
"var a = null;" +
"/** @type null */" +
"var b = a ? null : a;");
}
public void testHookRestrictsType3() throws Exception {
testTypes("/** @type {String} */" +
"var a;" +
"/** @type null */" +
"var b = (!a) ? a : null;");
}
public void testHookRestrictsType4() throws Exception {
testTypes("/** @type {(boolean,undefined)} */" +
"var a;" +
"/** @type boolean */" +
"var b = a != null ? a : true;");
}
public void testHookRestrictsType5() throws Exception {
testTypes("/** @type {(boolean,undefined)} */" +
"var a;" +
"/** @type {(undefined)} */" +
"var b = a == null ? a : undefined;");
}
public void testHookRestrictsType6() throws Exception {
testTypes("/** @type {(number,null,undefined)} */" +
"var a;" +
"/** @type {number} */" +
"var b = a == null ? 5 : a;");
}
public void testHookRestrictsType7() throws Exception {
testTypes("/** @type {(number,null,undefined)} */" +
"var a;" +
"/** @type {number} */" +
"var b = a == undefined ? 5 : a;");
}
public void testWhileRestrictsType1() throws Exception {
testTypes("/** @param {null} x */ function g(x) {}" +
"/** @param {number?} x */\n" +
"function f(x) {\n" +
"while (x) {\n" +
"if (g(x)) { x = 1; }\n" +
"x = x-1;\n}\n}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : number\n" +
"required: null");
}
public void testWhileRestrictsType2() throws Exception {
testTypes("/** @param {number?} x\n@return {number}*/\n" +
"function f(x) {\n/** @type {number} */var y = 0;" +
"while (x) {\n" +
"y = x;\n" +
"x = x-1;\n}\n" +
"return y;}");
}
public void testHigherOrderFunctions1() throws Exception {
testTypes(
"/** @type {function(number)} */var f;" +
"f(true);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testHigherOrderFunctions2() throws Exception {
testTypes(
"/** @type {function():!Date} */var f;" +
"/** @type boolean */var a = f();",
"initializing variable\n" +
"found : Date\n" +
"required: boolean");
}
public void testHigherOrderFunctions3() throws Exception {
testTypes(
"/** @type {function(this:Error):Date} */var f; new f",
"cannot instantiate non-constructor");
}
public void testHigherOrderFunctions4() throws Exception {
testTypes(
"/** @type {function(this:Error,...[number]):Date} */var f; new f",
"cannot instantiate non-constructor");
}
public void testHigherOrderFunctions5() throws Exception {
testTypes(
"/** @param {number} x */ function g(x) {}" +
"/** @type {function(new:Error,...[number]):Date} */ var f;" +
"g(new f());",
"actual parameter 1 of g does not match formal parameter\n" +
"found : Error\n" +
"required: number");
}
public void testConstructorAlias1() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {};" +
"/** @type {number} */ Foo.prototype.bar = 3;" +
"/** @constructor */ var FooAlias = Foo;" +
"/** @return {string} */ function foo() { " +
" return (new FooAlias()).bar; }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testConstructorAlias2() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {};" +
"/** @constructor */ var FooAlias = Foo;" +
"/** @type {number} */ FooAlias.prototype.bar = 3;" +
"/** @return {string} */ function foo() { " +
" return (new Foo()).bar; }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testConstructorAlias3() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {};" +
"/** @type {number} */ Foo.prototype.bar = 3;" +
"/** @constructor */ var FooAlias = Foo;" +
"/** @return {string} */ function foo() { " +
" return (new FooAlias()).bar; }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testConstructorAlias4() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {};" +
"var FooAlias = Foo;" +
"/** @type {number} */ FooAlias.prototype.bar = 3;" +
"/** @return {string} */ function foo() { " +
" return (new Foo()).bar; }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testConstructorAlias5() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {};" +
"/** @constructor */ var FooAlias = Foo;" +
"/** @return {FooAlias} */ function foo() { " +
" return new Foo(); }");
}
public void testConstructorAlias6() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {};" +
"/** @constructor */ var FooAlias = Foo;" +
"/** @return {Foo} */ function foo() { " +
" return new FooAlias(); }");
}
public void testConstructorAlias7() throws Exception {
testTypes(
"var goog = {};" +
"/** @constructor */ goog.Foo = function() {};" +
"/** @constructor */ goog.FooAlias = goog.Foo;" +
"/** @return {number} */ function foo() { " +
" return new goog.FooAlias(); }",
"inconsistent return type\n" +
"found : goog.Foo\n" +
"required: number");
}
public void testConstructorAlias8() throws Exception {
testTypes(
"var goog = {};" +
"/**\n * @param {number} x \n * @constructor */ " +
"goog.Foo = function(x) {};" +
"/**\n * @param {number} x \n * @constructor */ " +
"goog.FooAlias = goog.Foo;" +
"/** @return {number} */ function foo() { " +
" return new goog.FooAlias(1); }",
"inconsistent return type\n" +
"found : goog.Foo\n" +
"required: number");
}
public void testConstructorAlias9() throws Exception {
testTypes(
"var goog = {};" +
"/**\n * @param {number} x \n * @constructor */ " +
"goog.Foo = function(x) {};" +
"/** @constructor */ goog.FooAlias = goog.Foo;" +
"/** @return {number} */ function foo() { " +
" return new goog.FooAlias(1); }",
"inconsistent return type\n" +
"found : goog.Foo\n" +
"required: number");
}
public void testConstructorAlias10() throws Exception {
testTypes(
"/**\n * @param {number} x \n * @constructor */ " +
"var Foo = function(x) {};" +
"/** @constructor */ var FooAlias = Foo;" +
"/** @return {number} */ function foo() { " +
" return new FooAlias(1); }",
"inconsistent return type\n" +
"found : Foo\n" +
"required: number");
}
public void testClosure1() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {string|undefined} */var a;" +
"/** @type string */" +
"var b = goog.isDef(a) ? a : 'default';",
null);
}
public void testClosure2() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {string?} */var a;" +
"/** @type string */" +
"var b = goog.isNull(a) ? 'default' : a;",
null);
}
public void testClosure3() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {string|null|undefined} */var a;" +
"/** @type string */" +
"var b = goog.isDefAndNotNull(a) ? a : 'default';",
null);
}
public void testClosure4() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {string|undefined} */var a;" +
"/** @type string */" +
"var b = !goog.isDef(a) ? 'default' : a;",
null);
}
public void testClosure5() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {string?} */var a;" +
"/** @type string */" +
"var b = !goog.isNull(a) ? a : 'default';",
null);
}
public void testClosure6() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {string|null|undefined} */var a;" +
"/** @type string */" +
"var b = !goog.isDefAndNotNull(a) ? 'default' : a;",
null);
}
public void testClosure7() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {string|null|undefined} */ var a = foo();" +
"/** @type {number} */" +
"var b = goog.asserts.assert(a);",
"initializing variable\n" +
"found : string\n" +
"required: number");
}
public void testReturn1() throws Exception {
testTypes("/**@return {void}*/function foo(){ return 3; }",
"inconsistent return type\n" +
"found : number\n" +
"required: undefined");
}
public void testReturn2() throws Exception {
testTypes("/**@return {!Number}*/function foo(){ return; }",
"inconsistent return type\n" +
"found : undefined\n" +
"required: Number");
}
public void testReturn3() throws Exception {
testTypes("/**@return {!Number}*/function foo(){ return 'abc'; }",
"inconsistent return type\n" +
"found : string\n" +
"required: Number");
}
public void testReturn4() throws Exception {
testTypes("/**@return {!Number}\n*/\n function a(){return new Array();}",
"inconsistent return type\n" +
"found : Array\n" +
"required: Number");
}
public void testReturn5() throws Exception {
testTypes("/** @param {number} n\n" +
"@constructor */function n(n){return};");
}
public void testReturn6() throws Exception {
testTypes(
"/** @param {number} opt_a\n@return {string} */" +
"function a(opt_a) { return opt_a }",
"inconsistent return type\n" +
"found : (number|undefined)\n" +
"required: string");
}
public void testReturn7() throws Exception {
testTypes("/** @constructor */var A = function() {};\n" +
"/** @constructor */var B = function() {};\n" +
"/** @return {!B} */A.f = function() { return 1; };",
"inconsistent return type\n" +
"found : number\n" +
"required: B");
}
public void testReturn8() throws Exception {
testTypes("/** @constructor */var A = function() {};\n" +
"/** @constructor */var B = function() {};\n" +
"/** @return {!B} */A.prototype.f = function() { return 1; };",
"inconsistent return type\n" +
"found : number\n" +
"required: B");
}
public void testInferredReturn1() throws Exception {
testTypes(
"function f() {} /** @param {number} x */ function g(x) {}" +
"g(f());",
"actual parameter 1 of g does not match formal parameter\n" +
"found : undefined\n" +
"required: number");
}
public void testInferredReturn2() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() {}; " +
"/** @param {number} x */ function g(x) {}" +
"g((new Foo()).bar());",
"actual parameter 1 of g does not match formal parameter\n" +
"found : undefined\n" +
"required: number");
}
public void testInferredReturn3() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() {}; " +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @return {number} \n * @override */ " +
"SubFoo.prototype.bar = function() { return 3; }; ",
"mismatch of the bar property type and the type of the property " +
"it overrides from superclass Foo\n" +
"original: function (this:Foo): undefined\n" +
"override: function (this:SubFoo): number");
}
public void testInferredReturn4() throws Exception {
// By design, this throws a warning. if you want global x to be
// defined to some other type of function, then you need to declare it
// as a greater type.
testTypes(
"var x = function() {};" +
"x = /** @type {function(): number} */ (function() { return 3; });",
"assignment\n" +
"found : function (): number\n" +
"required: function (): undefined");
}
public void testInferredReturn5() throws Exception {
// If x is local, then the function type is not declared.
testTypes(
"/** @return {string} */" +
"function f() {" +
" var x = function() {};" +
" x = /** @type {function(): number} */ (function() { return 3; });" +
" return x();" +
"}",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testInferredReturn6() throws Exception {
testTypes(
"/** @return {string} */" +
"function f() {" +
" var x = function() {};" +
" if (f()) " +
" x = /** @type {function(): number} */ " +
" (function() { return 3; });" +
" return x();" +
"}",
"inconsistent return type\n" +
"found : (number|undefined)\n" +
"required: string");
}
public void testInferredReturn7() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @param {number} x */ Foo.prototype.bar = function(x) {};" +
"Foo.prototype.bar = function(x) { return 3; };",
"inconsistent return type\n" +
"found : number\n" +
"required: undefined");
}
public void testInferredReturn8() throws Exception {
reportMissingOverrides = CheckLevel.OFF;
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @param {number} x */ Foo.prototype.bar = function(x) {};" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @param {number} x */ SubFoo.prototype.bar = " +
" function(x) { return 3; }",
"inconsistent return type\n" +
"found : number\n" +
"required: undefined");
}
public void testInferredParam1() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @param {number} x */ Foo.prototype.bar = function(x) {};" +
"/** @param {string} x */ function f(x) {}" +
"Foo.prototype.bar = function(y) { f(y); };",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testInferredParam2() throws Exception {
reportMissingOverrides = CheckLevel.OFF;
testTypes(
"/** @param {string} x */ function f(x) {}" +
"/** @constructor */ function Foo() {}" +
"/** @param {number} x */ Foo.prototype.bar = function(x) {};" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @return {void} */ SubFoo.prototype.bar = " +
" function(x) { f(x); }",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testInferredParam3() throws Exception {
reportMissingOverrides = CheckLevel.OFF;
testTypes(
"/** @param {string} x */ function f(x) {}" +
"/** @constructor */ function Foo() {}" +
"/** @param {number=} x */ Foo.prototype.bar = function(x) {};" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @return {void} */ SubFoo.prototype.bar = " +
" function(x) { f(x); }; (new SubFoo()).bar();",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (number|undefined)\n" +
"required: string");
}
public void testInferredParam4() throws Exception {
reportMissingOverrides = CheckLevel.OFF;
testTypes(
"/** @param {string} x */ function f(x) {}" +
"/** @constructor */ function Foo() {}" +
"/** @param {...number} x */ Foo.prototype.bar = function(x) {};" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @return {void} */ SubFoo.prototype.bar = " +
" function(x) { f(x); }; (new SubFoo()).bar();",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (number|undefined)\n" +
"required: string");
}
public void testInferredParam5() throws Exception {
reportMissingOverrides = CheckLevel.OFF;
testTypes(
"/** @param {string} x */ function f(x) {}" +
"/** @constructor */ function Foo() {}" +
"/** @param {...number} x */ Foo.prototype.bar = function(x) {};" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @param {number=} x \n * @param {...number} y */ " +
"SubFoo.prototype.bar = " +
" function(x, y) { f(x); }; (new SubFoo()).bar();",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (number|undefined)\n" +
"required: string");
}
public void testInferredParam6() throws Exception {
reportMissingOverrides = CheckLevel.OFF;
testTypes(
"/** @param {string} x */ function f(x) {}" +
"/** @constructor */ function Foo() {}" +
"/** @param {number=} x */ Foo.prototype.bar = function(x) {};" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @param {number=} x \n * @param {number=} y */ " +
"SubFoo.prototype.bar = " +
" function(x, y) { f(y); };",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (number|undefined)\n" +
"required: string");
}
public void testOverriddenParams1() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @param {...?} var_args */" +
"Foo.prototype.bar = function(var_args) {};" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/**\n" +
" * @param {number} x\n" +
" * @override\n" +
" */" +
"SubFoo.prototype.bar = function(x) {};");
}
public void testOverriddenParams2() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @type {function(...[?])} */" +
"Foo.prototype.bar = function(var_args) {};" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/**\n" +
" * @type {function(number)}\n" +
" * @override\n" +
" */" +
"SubFoo.prototype.bar = function(x) {};");
}
public void testOverriddenParams3() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @param {...number} var_args */" +
"Foo.prototype.bar = function(var_args) { };" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/**\n" +
" * @param {number} x\n" +
" * @override\n" +
" */" +
"SubFoo.prototype.bar = function(x) {};",
"mismatch of the bar property type and the type of the " +
"property it overrides from superclass Foo\n" +
"original: function (this:Foo, ...[number]): undefined\n" +
"override: function (this:SubFoo, number): undefined");
}
public void testOverriddenParams4() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @type {function(...[number])} */" +
"Foo.prototype.bar = function(var_args) {};" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/**\n" +
" * @type {function(number)}\n" +
" * @override\n" +
" */" +
"SubFoo.prototype.bar = function(x) {};",
"mismatch of the bar property type and the type of the " +
"property it overrides from superclass Foo\n" +
"original: function (...[number]): ?\n" +
"override: function (number): ?");
}
public void testOverriddenParams5() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @param {number} x */" +
"Foo.prototype.bar = function(x) { };" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/**\n" +
" * @override\n" +
" */" +
"SubFoo.prototype.bar = function() {};" +
"(new SubFoo()).bar();");
}
public void testOverriddenParams6() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @param {number} x */" +
"Foo.prototype.bar = function(x) { };" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/**\n" +
" * @override\n" +
" */" +
"SubFoo.prototype.bar = function() {};" +
"(new SubFoo()).bar(true);",
"actual parameter 1 of SubFoo.prototype.bar " +
"does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testOverriddenReturn1() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @return {Object} */ Foo.prototype.bar = " +
" function() { return {}; };" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @return {SubFoo}\n * @override */ SubFoo.prototype.bar = " +
" function() { return new Foo(); }",
"inconsistent return type\n" +
"found : Foo\n" +
"required: (SubFoo|null)");
}
public void testOverriddenReturn2() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @return {SubFoo} */ Foo.prototype.bar = " +
" function() { return new SubFoo(); };" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @return {Foo} x\n * @override */ SubFoo.prototype.bar = " +
" function() { return new SubFoo(); }",
"mismatch of the bar property type and the type of the " +
"property it overrides from superclass Foo\n" +
"original: function (this:Foo): (SubFoo|null)\n" +
"override: function (this:SubFoo): (Foo|null)");
}
public void testThis1() throws Exception {
testTypes("var goog = {};" +
"/** @constructor */goog.A = function(){};" +
"/** @return {number} */" +
"goog.A.prototype.n = function() { return this };",
"inconsistent return type\n" +
"found : goog.A\n" +
"required: number");
}
public void testOverriddenProperty1() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @type {Object} */" +
"Foo.prototype.bar = {};" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/**\n" +
" * @type {Array}\n" +
" * @override\n" +
" */" +
"SubFoo.prototype.bar = [];");
}
public void testOverriddenProperty2() throws Exception {
testTypes(
"/** @constructor */ function Foo() {" +
" /** @type {Object} */" +
" this.bar = {};" +
"}" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/**\n" +
" * @type {Array}\n" +
" * @override\n" +
" */" +
"SubFoo.prototype.bar = [];");
}
public void testThis2() throws Exception {
testTypes("var goog = {};" +
"/** @constructor */goog.A = function(){" +
" this.foo = null;" +
"};" +
"/** @return {number} */" +
"goog.A.prototype.n = function() { return this.foo };",
"inconsistent return type\n" +
"found : null\n" +
"required: number");
}
public void testThis3() throws Exception {
testTypes("var goog = {};" +
"/** @constructor */goog.A = function(){" +
" this.foo = null;" +
" this.foo = 5;" +
"};");
}
public void testThis4() throws Exception {
testTypes("var goog = {};" +
"/** @constructor */goog.A = function(){" +
" /** @type {string?} */this.foo = null;" +
"};" +
"/** @return {number} */goog.A.prototype.n = function() {" +
" return this.foo };",
"inconsistent return type\n" +
"found : (null|string)\n" +
"required: number");
}
public void testThis5() throws Exception {
testTypes("/** @this Date\n@return {number}*/function h() { return this }",
"inconsistent return type\n" +
"found : Date\n" +
"required: number");
}
public void testThis6() throws Exception {
testTypes("var goog = {};" +
"/** @constructor\n@return {!Date} */" +
"goog.A = function(){ return this };",
"inconsistent return type\n" +
"found : goog.A\n" +
"required: Date");
}
public void testThis7() throws Exception {
testTypes("/** @constructor */function A(){};" +
"/** @return {number} */A.prototype.n = function() { return this };",
"inconsistent return type\n" +
"found : A\n" +
"required: number");
}
public void testThis8() throws Exception {
testTypes("/** @constructor */function A(){" +
" /** @type {string?} */this.foo = null;" +
"};" +
"/** @return {number} */A.prototype.n = function() {" +
" return this.foo };",
"inconsistent return type\n" +
"found : (null|string)\n" +
"required: number");
}
public void testThis9() throws Exception {
// In A.bar, the type of {@code this} is unknown.
testTypes("/** @constructor */function A(){};" +
"A.prototype.foo = 3;" +
"/** @return {string} */ A.bar = function() { return this.foo; };");
}
public void testThis10() throws Exception {
// In A.bar, the type of {@code this} is inferred from the @this tag.
testTypes("/** @constructor */function A(){};" +
"A.prototype.foo = 3;" +
"/** @this {A}\n@return {string} */" +
"A.bar = function() { return this.foo; };",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testThis11() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"/** @constructor */ function Ctor() {" +
" /** @this {Date} */" +
" this.method = function() {" +
" f(this);" +
" };" +
"}",
"actual parameter 1 of f does not match formal parameter\n" +
"found : Date\n" +
"required: number");
}
public void testThis12() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"/** @constructor */ function Ctor() {}" +
"Ctor.prototype['method'] = function() {" +
" f(this);" +
"}",
"actual parameter 1 of f does not match formal parameter\n" +
"found : Ctor\n" +
"required: number");
}
public void testThis13() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"/** @constructor */ function Ctor() {}" +
"Ctor.prototype = {" +
" method: function() {" +
" f(this);" +
" }" +
"};",
"actual parameter 1 of f does not match formal parameter\n" +
"found : Ctor\n" +
"required: number");
}
public void testThis14() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"f(this.Object);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : function (new:Object, *=): ?\n" +
"required: number");
}
public void testThisTypeOfFunction1() throws Exception {
testTypes(
"/** @type {function(this:Object)} */ function f() {}" +
"f();");
}
public void testThisTypeOfFunction2() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** @type {function(this:F)} */ function f() {}" +
"f();",
"\"function (this:F): ?\" must be called with a \"this\" type");
}
public void testThisTypeOfFunction3() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"F.prototype.bar = function() {};" +
"var f = (new F()).bar; f();",
"\"function (this:F): undefined\" must be called with a \"this\" type");
}
public void testThisTypeOfFunction4() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"F.prototype.moveTo = function(x, y) {};" +
"F.prototype.lineTo = function(x, y) {};" +
"function demo() {" +
" var path = new F();" +
" var points = [[1,1], [2,2]];" +
" for (var i = 0; i < points.length; i++) {" +
" (i == 0 ? path.moveTo : path.lineTo)(" +
" points[i][0], points[i][1]);" +
" }" +
"}",
"\"function (this:F, ?, ?): undefined\" " +
"must be called with a \"this\" type");
}
public void testGlobalThis1() throws Exception {
testTypes("/** @constructor */ function Window() {}" +
"/** @param {string} msg */ " +
"Window.prototype.alert = function(msg) {};" +
"this.alert(3);",
"actual parameter 1 of Window.prototype.alert " +
"does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testGlobalThis2() throws Exception {
// this.alert = 3 doesn't count as a declaration, so this isn't a warning.
testTypes("/** @constructor */ function Bindow() {}" +
"/** @param {string} msg */ " +
"Bindow.prototype.alert = function(msg) {};" +
"this.alert = 3;" +
"(new Bindow()).alert(this.alert)");
}
public void testGlobalThis2b() throws Exception {
testTypes("/** @constructor */ function Bindow() {}" +
"/** @param {string} msg */ " +
"Bindow.prototype.alert = function(msg) {};" +
"/** @return {number} */ this.alert = function() { return 3; };" +
"(new Bindow()).alert(this.alert())",
"actual parameter 1 of Bindow.prototype.alert " +
"does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testGlobalThis3() throws Exception {
testTypes(
"/** @param {string} msg */ " +
"function alert(msg) {};" +
"this.alert(3);",
"actual parameter 1 of global this.alert " +
"does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testGlobalThis4() throws Exception {
testTypes(
"/** @param {string} msg */ " +
"var alert = function(msg) {};" +
"this.alert(3);",
"actual parameter 1 of global this.alert " +
"does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testGlobalThis5() throws Exception {
testTypes(
"function f() {" +
" /** @param {string} msg */ " +
" var alert = function(msg) {};" +
"}" +
"this.alert(3);",
"Property alert never defined on global this");
}
public void testGlobalThis6() throws Exception {
testTypes(
"/** @param {string} msg */ " +
"var alert = function(msg) {};" +
"var x = 3;" +
"x = 'msg';" +
"this.alert(this.x);");
}
public void testGlobalThis7() throws Exception {
testTypes(
"/** @constructor */ function Window() {}" +
"/** @param {Window} msg */ " +
"var foo = function(msg) {};" +
"foo(this);");
}
public void testGlobalThis8() throws Exception {
testTypes(
"/** @constructor */ function Window() {}" +
"/** @param {number} msg */ " +
"var foo = function(msg) {};" +
"foo(this);",
"actual parameter 1 of foo does not match formal parameter\n" +
"found : global this\n" +
"required: number");
}
public void testGlobalThis9() throws Exception {
testTypes(
// Window is not marked as a constructor, so the
// inheritance doesn't happen.
"function Window() {}" +
"Window.prototype.alert = function() {};" +
"this.alert();",
"Property alert never defined on global this");
}
public void testControlFlowRestrictsType1() throws Exception {
testTypes("/** @return {String?} */ function f() { return null; }" +
"/** @type {String?} */ var a = f();" +
"/** @type String */ var b = new String('foo');" +
"/** @type null */ var c = null;" +
"if (a) {" +
" b = a;" +
"} else {" +
" c = a;" +
"}");
}
public void testControlFlowRestrictsType2() throws Exception {
testTypes("/** @return {(string,null)} */ function f() { return null; }" +
"/** @type {(string,null)} */ var a = f();" +
"/** @type string */ var b = 'foo';" +
"/** @type null */ var c = null;" +
"if (a) {" +
" b = a;" +
"} else {" +
" c = a;" +
"}",
"assignment\n" +
"found : (null|string)\n" +
"required: null");
}
public void testControlFlowRestrictsType3() throws Exception {
testTypes("/** @type {(string,void)} */" +
"var a;" +
"/** @type string */" +
"var b = 'foo';" +
"if (a) {" +
" b = a;" +
"}");
}
public void testControlFlowRestrictsType4() throws Exception {
testTypes("/** @param {string} a */ function f(a){}" +
"/** @type {(string,undefined)} */ var a;" +
"a && f(a);");
}
public void testControlFlowRestrictsType5() throws Exception {
testTypes("/** @param {undefined} a */ function f(a){}" +
"/** @type {(!Array,undefined)} */ var a;" +
"a || f(a);");
}
public void testControlFlowRestrictsType6() throws Exception {
testTypes("/** @param {undefined} x */ function f(x) {}" +
"/** @type {(string,undefined)} */ var a;" +
"a && f(a);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: undefined");
}
public void testControlFlowRestrictsType7() throws Exception {
testTypes("/** @param {undefined} x */ function f(x) {}" +
"/** @type {(string,undefined)} */ var a;" +
"a && f(a);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: undefined");
}
public void testControlFlowRestrictsType8() throws Exception {
testTypes("/** @param {undefined} a */ function f(a){}" +
"/** @type {(!Array,undefined)} */ var a;" +
"if (a || f(a)) {}");
}
public void testControlFlowRestrictsType9() throws Exception {
testTypes("/** @param {number?} x\n * @return {number}*/\n" +
"var f = function(x) {\n" +
"if (!x || x == 1) { return 1; } else { return x; }\n" +
"};");
}
public void testControlFlowRestrictsType10() throws Exception {
// We should correctly infer that y will be (null|{}) because
// the loop wraps around.
testTypes("/** @param {number} x */ function f(x) {}" +
"function g() {" +
" var y = null;" +
" for (var i = 0; i < 10; i++) {" +
" f(y);" +
" if (y != null) {" +
" // y is None the first time it goes thru this branch\n" +
" } else {" +
" y = {};" +
" }" +
" }" +
"};",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (null|{})\n" +
"required: number");
}
public void testControlFlowRestrictsType11() throws Exception {
testTypes("/** @param {boolean} x */ function f(x) {}" +
"function g() {" +
" var y = null;" +
" if (y != null) {" +
" for (var i = 0; i < 10; i++) {" +
" f(y);" +
" }" +
" }" +
"};",
"condition always evaluates to false\n" +
"left : null\n" +
"right: null");
}
public void testSwitchCase3() throws Exception {
testTypes("/** @type String */" +
"var a = new String('foo');" +
"switch (a) { case 'A': }");
}
public void testSwitchCase4() throws Exception {
testTypes("/** @type {(string,Null)} */" +
"var a = 'foo';" +
"switch (a) { case 'A':break; case null:break; }");
}
public void testSwitchCase5() throws Exception {
testTypes("/** @type {(String,Null)} */" +
"var a = new String('foo');" +
"switch (a) { case 'A':break; case null:break; }");
}
public void testSwitchCase6() throws Exception {
testTypes("/** @type {(Number,Null)} */" +
"var a = new Number(5);" +
"switch (a) { case 5:break; case null:break; }");
}
public void testSwitchCase7() throws Exception {
// This really tests the inference inside the case.
testTypes(
"/**\n" +
" * @param {number} x\n" +
" * @return {number}\n" +
" */\n" +
"function g(x) { return 5; }" +
"function f() {" +
" var x = {};" +
" x.foo = '3';" +
" switch (3) { case g(x.foo): return 3; }" +
"}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testSwitchCase8() throws Exception {
// This really tests the inference inside the switch clause.
testTypes(
"/**\n" +
" * @param {number} x\n" +
" * @return {number}\n" +
" */\n" +
"function g(x) { return 5; }" +
"function f() {" +
" var x = {};" +
" x.foo = '3';" +
" switch (g(x.foo)) { case 3: return 3; }" +
"}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testNoTypeCheck1() throws Exception {
testTypes("/** @notypecheck */function foo() { new 4 }");
}
public void testNoTypeCheck2() throws Exception {
testTypes("/** @notypecheck */var foo = function() { new 4 }");
}
public void testNoTypeCheck3() throws Exception {
testTypes("/** @notypecheck */var foo = function bar() { new 4 }");
}
public void testNoTypeCheck4() throws Exception {
testTypes("var foo;" +
"/** @notypecheck */foo = function() { new 4 }");
}
public void testNoTypeCheck5() throws Exception {
testTypes("var foo;" +
"foo = /** @notypecheck */function() { new 4 }");
}
public void testNoTypeCheck6() throws Exception {
testTypes("var foo;" +
"/** @notypecheck */foo = function bar() { new 4 }");
}
public void testNoTypeCheck7() throws Exception {
testTypes("var foo;" +
"foo = /** @notypecheck */function bar() { new 4 }");
}
public void testNoTypeCheck8() throws Exception {
testTypes("/** @fileoverview \n * @notypecheck */ var foo;" +
"var bar = 3; /** @param {string} x */ function f(x) {} f(bar);");
}
public void testNoTypeCheck9() throws Exception {
testTypes("/** @notypecheck */ function g() { }" +
" /** @type {string} */ var a = 1",
"initializing variable\n" +
"found : number\n" +
"required: string"
);
}
public void testNoTypeCheck10() throws Exception {
testTypes("/** @notypecheck */ function g() { }" +
" function h() {/** @type {string} */ var a = 1}",
"initializing variable\n" +
"found : number\n" +
"required: string"
);
}
public void testNoTypeCheck11() throws Exception {
testTypes("/** @notypecheck */ function g() { }" +
"/** @notypecheck */ function h() {/** @type {string} */ var a = 1}"
);
}
public void testNoTypeCheck12() throws Exception {
testTypes("/** @notypecheck */ function g() { }" +
"function h() {/** @type {string}\n * @notypecheck\n*/ var a = 1}"
);
}
public void testNoTypeCheck13() throws Exception {
testTypes("/** @notypecheck */ function g() { }" +
"function h() {/** @type {string}\n * @notypecheck\n*/ var a = 1;" +
"/** @type {string}*/ var b = 1}",
"initializing variable\n" +
"found : number\n" +
"required: string"
);
}
public void testNoTypeCheck14() throws Exception {
testTypes("/** @fileoverview \n * @notypecheck */ function g() { }" +
"g(1,2,3)");
}
public void testImplicitCast() throws Exception {
testTypes("/** @constructor */ function Element() {};\n" +
"/** @type {string}\n" +
" * @implicitCast */" +
"Element.prototype.innerHTML;",
"(new Element).innerHTML = new Array();", null, false);
}
public void testImplicitCastSubclassAccess() throws Exception {
testTypes("/** @constructor */ function Element() {};\n" +
"/** @type {string}\n" +
" * @implicitCast */" +
"Element.prototype.innerHTML;" +
"/** @constructor \n @extends Element */" +
"function DIVElement() {};",
"(new DIVElement).innerHTML = new Array();",
null, false);
}
public void testImplicitCastNotInExterns() throws Exception {
testTypes("/** @constructor */ function Element() {};\n" +
"/** @type {string}\n" +
" * @implicitCast */" +
"Element.prototype.innerHTML;" +
"(new Element).innerHTML = new Array();",
new String[] {
"Illegal annotation on innerHTML. @implicitCast may only be " +
"used in externs.",
"assignment to property innerHTML of Element\n" +
"found : Array\n" +
"required: string"});
}
public void testNumberNode() throws Exception {
Node n = typeCheck(Node.newNumber(0));
assertEquals(NUMBER_TYPE, n.getJSType());
}
public void testStringNode() throws Exception {
Node n = typeCheck(Node.newString("hello"));
assertEquals(STRING_TYPE, n.getJSType());
}
public void testBooleanNodeTrue() throws Exception {
Node trueNode = typeCheck(new Node(Token.TRUE));
assertEquals(BOOLEAN_TYPE, trueNode.getJSType());
}
public void testBooleanNodeFalse() throws Exception {
Node falseNode = typeCheck(new Node(Token.FALSE));
assertEquals(BOOLEAN_TYPE, falseNode.getJSType());
}
public void testUndefinedNode() throws Exception {
Node p = new Node(Token.ADD);
Node n = Node.newString(Token.NAME, "undefined");
p.addChildToBack(n);
p.addChildToBack(Node.newNumber(5));
typeCheck(p);
assertEquals(VOID_TYPE, n.getJSType());
}
public void testNumberAutoboxing() throws Exception {
testTypes("/** @type Number */var a = 4;",
"initializing variable\n" +
"found : number\n" +
"required: (Number|null)");
}
public void testNumberUnboxing() throws Exception {
testTypes("/** @type number */var a = new Number(4);",
"initializing variable\n" +
"found : Number\n" +
"required: number");
}
public void testStringAutoboxing() throws Exception {
testTypes("/** @type String */var a = 'hello';",
"initializing variable\n" +
"found : string\n" +
"required: (String|null)");
}
public void testStringUnboxing() throws Exception {
testTypes("/** @type string */var a = new String('hello');",
"initializing variable\n" +
"found : String\n" +
"required: string");
}
public void testBooleanAutoboxing() throws Exception {
testTypes("/** @type Boolean */var a = true;",
"initializing variable\n" +
"found : boolean\n" +
"required: (Boolean|null)");
}
public void testBooleanUnboxing() throws Exception {
testTypes("/** @type boolean */var a = new Boolean(false);",
"initializing variable\n" +
"found : Boolean\n" +
"required: boolean");
}
public void testIssue86() throws Exception {
testTypes(
"/** @interface */ function I() {}" +
"/** @return {number} */ I.prototype.get = function(){};" +
"/** @constructor \n * @implements {I} */ function F() {}" +
"/** @override */ F.prototype.get = function() { return true; };",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testIssue124() throws Exception {
testTypes(
"var t = null;" +
"function test() {" +
" if (t != null) { t = null; }" +
" t = 1;" +
"}");
}
public void testIssue124b() throws Exception {
testTypes(
"var t = null;" +
"function test() {" +
" if (t != null) { t = null; }" +
" t = undefined;" +
"}",
"condition always evaluates to false\n" +
"left : (null|undefined)\n" +
"right: null");
}
public void testIssue259() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"/** @constructor */" +
"var Clock = function() {" +
" /** @constructor */" +
" this.Date = function() {};" +
" f(new this.Date());" +
"};",
"actual parameter 1 of f does not match formal parameter\n" +
"found : this.Date\n" +
"required: number");
}
public void testIssue301() throws Exception {
testTypes(
"Array.indexOf = function() {};" +
"var s = 'hello';" +
"alert(s.toLowerCase.indexOf('1'));",
"Property indexOf never defined on String.prototype.toLowerCase");
}
public void testIssue368() throws Exception {
testTypes(
"/** @constructor */ function Foo(){}" +
"/**\n" +
" * @param {number} one\n" +
" * @param {string} two\n" +
" */\n" +
"Foo.prototype.add = function(one, two) {};" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */\n" +
"function Bar(){}" +
"/** @override */\n" +
"Bar.prototype.add = function(ignored) {};" +
"(new Bar()).add(1, 2);",
"actual parameter 2 of Bar.prototype.add does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testIssue380() throws Exception {
testTypes(
"/** @type { function(string): {innerHTML: string} } */" +
"document.getElementById;" +
"var list = /** @type {!Array.<string>} */ ['hello', 'you'];\n" +
"list.push('?');\n" +
"document.getElementById('node').innerHTML = list.toString();");
}
public void testIssue483() throws Exception {
testTypes(
"/** @constructor */ function C() {" +
" /** @type {?Array} */ this.a = [];" +
"}" +
"C.prototype.f = function() {" +
" if (this.a.length > 0) {" +
" g(this.a);" +
" }" +
"};" +
"/** @param {number} a */ function g(a) {}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : Array\n" +
"required: number");
}
public void testIssue537a() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype = {method: function() {}};" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */\n" +
"function Bar() {" +
" Foo.call(this);" +
" if (this.baz()) this.method(1);" +
"}" +
"Bar.prototype = {" +
" baz: function() {" +
" return true;" +
" }" +
"};" +
"Bar.prototype.__proto__ = Foo.prototype;",
"Function Foo.prototype.method: called with 1 argument(s). " +
"Function requires at least 0 argument(s) " +
"and no more than 0 argument(s).");
}
public void testIssue537b() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype = {method: function() {}};" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */\n" +
"function Bar() {" +
" Foo.call(this);" +
" if (this.baz(1)) this.method();" +
"}" +
"Bar.prototype = {" +
" baz: function() {" +
" return true;" +
" }" +
"};" +
"Bar.prototype.__proto__ = Foo.prototype;",
"Function Bar.prototype.baz: called with 1 argument(s). " +
"Function requires at least 0 argument(s) " +
"and no more than 0 argument(s).");
}
public void testIssue537c() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */\n" +
"function Bar() {" +
" Foo.call(this);" +
" if (this.baz2()) alert(1);" +
"}" +
"Bar.prototype = {" +
" baz: function() {" +
" return true;" +
" }" +
"};" +
"Bar.prototype.__proto__ = Foo.prototype;",
"Property baz2 never defined on Bar");
}
public void testIssue537d() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype = {" +
" /** @return {Bar} */ x: function() { new Bar(); }," +
" /** @return {Foo} */ y: function() { new Bar(); }" +
"};" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */\n" +
"function Bar() {" +
" this.xy = 3;" +
"}" +
"/** @return {Bar} */ function f() { return new Bar(); }" +
"/** @return {Foo} */ function g() { return new Bar(); }" +
"Bar.prototype = {" +
" /** @return {Bar} */ x: function() { new Bar(); }," +
" /** @return {Foo} */ y: function() { new Bar(); }" +
"};" +
"Bar.prototype.__proto__ = Foo.prototype;");
}
public void testIssue586() throws Exception {
testTypes(
"/** @constructor */" +
"var MyClass = function() {};" +
"/** @param {boolean} success */" +
"MyClass.prototype.fn = function(success) {};" +
"MyClass.prototype.test = function() {" +
" this.fn();" +
" this.fn = function() {};" +
"};",
"Function MyClass.prototype.fn: called with 0 argument(s). " +
"Function requires at least 1 argument(s) " +
"and no more than 1 argument(s).");
}
public void testIssue635() throws Exception {
// TODO(nicksantos): Make this emit a warning, because of the 'this' type.
testTypes(
"/** @constructor */" +
"function F() {}" +
"F.prototype.bar = function() { this.baz(); };" +
"F.prototype.baz = function() {};" +
"/** @constructor */" +
"function G() {}" +
"G.prototype.bar = F.prototype.bar;");
}
public void testIssue669() throws Exception {
testTypes(
"/** @return {{prop1: (Object|undefined)}} */" +
"function f(a) {" +
" var results;" +
" if (a) {" +
" results = {};" +
" results.prop1 = {a: 3};" +
" } else {" +
" results = {prop2: 3};" +
" }" +
" return results;" +
"}");
}
public void testIssue700() throws Exception {
testTypes(
"/**\n" +
" * @param {{text: string}} opt_data\n" +
" * @return {string}\n" +
" */\n" +
"function temp1(opt_data) {\n" +
" return opt_data.text;\n" +
"}\n" +
"\n" +
"/**\n" +
" * @param {{activity: (boolean|number|string|null|Object)}} opt_data\n" +
" * @return {string}\n" +
" */\n" +
"function temp2(opt_data) {\n" +
" /** @notypecheck */\n" +
" function __inner() {\n" +
" return temp1(opt_data.activity);\n" +
" }\n" +
" return __inner();\n" +
"}\n" +
"\n" +
"/**\n" +
" * @param {{n: number, text: string, b: boolean}} opt_data\n" +
" * @return {string}\n" +
" */\n" +
"function temp3(opt_data) {\n" +
" return 'n: ' + opt_data.n + ', t: ' + opt_data.text + '.';\n" +
"}\n" +
"\n" +
"function callee() {\n" +
" var output = temp3({\n" +
" n: 0,\n" +
" text: 'a string',\n" +
" b: true\n" +
" })\n" +
" alert(output);\n" +
"}\n" +
"\n" +
"callee();");
}
/**
* Tests that the || operator is type checked correctly, that is of
* the type of the first argument or of the second argument. See
* bugid 592170 for more details.
*/
public void testBug592170() throws Exception {
testTypes(
"/** @param {Function} opt_f ... */" +
"function foo(opt_f) {" +
" /** @type {Function} */" +
" return opt_f || function () {};" +
"}");
}
/**
* Tests that undefined can be compared shallowly to a value of type
* (number,undefined) regardless of the side on which the undefined
* value is.
*/
public void testBug901455() throws Exception {
testTypes("/** @return {(number,undefined)} */ function a() { return 3; }" +
"var b = undefined === a()");
testTypes("/** @return {(number,undefined)} */ function a() { return 3; }" +
"var b = a() === undefined");
}
/**
* Tests that the match method of strings returns nullable arrays.
*/
public void testBug908701() throws Exception {
testTypes("/** @type {String} */var s = new String('foo');" +
"var b = s.match(/a/) != null;");
}
/**
* Tests that named types play nicely with subtyping.
*/
public void testBug908625() throws Exception {
testTypes("/** @constructor */function A(){}" +
"/** @constructor\n * @extends A */function B(){}" +
"/** @param {B} b" +
"\n @return {(A,undefined)} */function foo(b){return b}");
}
/**
* Tests that assigning two untyped functions to a variable whose type is
* inferred and calling this variable is legal.
*/
public void testBug911118() throws Exception {
// verifying the type assigned to function expressions assigned variables
Scope s = parseAndTypeCheckWithScope("var a = function(){};").scope;
JSType type = s.getVar("a").getType();
assertEquals("function (): undefined", type.toString());
// verifying the bug example
testTypes("function nullFunction() {};" +
"var foo = nullFunction;" +
"foo = function() {};" +
"foo();");
}
public void testBug909000() throws Exception {
testTypes("/** @constructor */function A(){}\n" +
"/** @param {!A} a\n" +
"@return {boolean}*/\n" +
"function y(a) { return a }",
"inconsistent return type\n" +
"found : A\n" +
"required: boolean");
}
public void testBug930117() throws Exception {
testTypes(
"/** @param {boolean} x */function f(x){}" +
"f(null);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : null\n" +
"required: boolean");
}
public void testBug1484445() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @type {number?} */ Foo.prototype.bar = null;" +
"/** @type {number?} */ Foo.prototype.baz = null;" +
"/** @param {Foo} foo */" +
"function f(foo) {" +
" while (true) {" +
" if (foo.bar == null && foo.baz == null) {" +
" foo.bar;" +
" }" +
" }" +
"}");
}
public void testBug1859535() throws Exception {
testTypes(
"/**\n" +
" * @param {Function} childCtor Child class.\n" +
" * @param {Function} parentCtor Parent class.\n" +
" */" +
"var inherits = function(childCtor, parentCtor) {" +
" /** @constructor */" +
" function tempCtor() {};" +
" tempCtor.prototype = parentCtor.prototype;" +
" childCtor.superClass_ = parentCtor.prototype;" +
" childCtor.prototype = new tempCtor();" +
" /** @override */ childCtor.prototype.constructor = childCtor;" +
"};" +
"/**" +
" * @param {Function} constructor\n" +
" * @param {Object} var_args\n" +
" * @return {Object}\n" +
" */" +
"var factory = function(constructor, var_args) {" +
" /** @constructor */" +
" var tempCtor = function() {};" +
" tempCtor.prototype = constructor.prototype;" +
" var obj = new tempCtor();" +
" constructor.apply(obj, arguments);" +
" return obj;" +
"};");
}
public void testBug1940591() throws Exception {
testTypes(
"/** @type {Object} */" +
"var a = {};\n" +
"/** @type {number} */\n" +
"a.name = 0;\n" +
"/**\n" +
" * @param {Function} x anything.\n" +
" */\n" +
"a.g = function(x) { x.name = 'a'; }");
}
public void testBug1942972() throws Exception {
testTypes(
"var google = {\n" +
" gears: {\n" +
" factory: {},\n" +
" workerPool: {}\n" +
" }\n" +
"};\n" +
"\n" +
"google.gears = {factory: {}};\n");
}
public void testBug1943776() throws Exception {
testTypes(
"/** @return {{foo: Array}} */" +
"function bar() {" +
" return {foo: []};" +
"}");
}
public void testBug1987544() throws Exception {
testTypes(
"/** @param {string} x */ function foo(x) {}" +
"var duration;" +
"if (true && !(duration = 3)) {" +
" foo(duration);" +
"}",
"actual parameter 1 of foo does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testBug1940769() throws Exception {
testTypes(
"/** @return {!Object} */ " +
"function proto(obj) { return obj.prototype; }" +
"/** @constructor */ function Map() {}" +
"/**\n" +
" * @constructor\n" +
" * @extends {Map}\n" +
" */" +
"function Map2() { Map.call(this); };" +
"Map2.prototype = proto(Map);");
}
public void testBug2335992() throws Exception {
testTypes(
"/** @return {*} */ function f() { return 3; }" +
"var x = f();" +
"/** @type {string} */" +
"x.y = 3;",
"assignment\n" +
"found : number\n" +
"required: string");
}
public void testBug2341812() throws Exception {
testTypes(
"/** @interface */" +
"function EventTarget() {}" +
"/** @constructor \n * @implements {EventTarget} */" +
"function Node() {}" +
"/** @type {number} */ Node.prototype.index;" +
"/** @param {EventTarget} x \n * @return {string} */" +
"function foo(x) { return x.index; }");
}
public void testScopedConstructors1() throws Exception {
testTypes(
"function foo1() { " +
" /** @constructor */ function Bar() { " +
" /** @type {number} */ this.x = 3;" +
" }" +
"}" +
"function foo2() { " +
" /** @constructor */ function Bar() { " +
" /** @type {string} */ this.x = 'y';" +
" }" +
" /** " +
" * @param {Bar} b\n" +
" * @return {number}\n" +
" */" +
" function baz(b) { return b.x; }" +
"}",
"inconsistent return type\n" +
"found : string\n" +
"required: number");
}
public void testScopedConstructors2() throws Exception {
testTypes(
"/** @param {Function} f */" +
"function foo1(f) {" +
" /** @param {Function} g */" +
" f.prototype.bar = function(g) {};" +
"}");
}
public void testQualifiedNameInference1() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @type {number?} */ Foo.prototype.bar = null;" +
"/** @type {number?} */ Foo.prototype.baz = null;" +
"/** @param {Foo} foo */" +
"function f(foo) {" +
" while (true) {" +
" if (!foo.baz) break; " +
" foo.bar = null;" +
" }" +
// Tests a bug where this condition always evaluated to true.
" return foo.bar == null;" +
"}");
}
public void testQualifiedNameInference2() throws Exception {
testTypes(
"var x = {};" +
"x.y = c;" +
"function f(a, b) {" +
" if (a) {" +
" if (b) " +
" x.y = 2;" +
" else " +
" x.y = 1;" +
" }" +
" return x.y == null;" +
"}");
}
public void testQualifiedNameInference3() throws Exception {
testTypes(
"var x = {};" +
"x.y = c;" +
"function f(a, b) {" +
" if (a) {" +
" if (b) " +
" x.y = 2;" +
" else " +
" x.y = 1;" +
" }" +
" return x.y == null;" +
"} function g() { x.y = null; }");
}
public void testQualifiedNameInference4() throws Exception {
testTypes(
"/** @param {string} x */ function f(x) {}\n" +
"/**\n" +
" * @param {?string} x \n" +
" * @constructor\n" +
" */" +
"function Foo(x) { this.x_ = x; }\n" +
"Foo.prototype.bar = function() {" +
" if (this.x_) { f(this.x_); }" +
"};");
}
public void testQualifiedNameInference5() throws Exception {
testTypes(
"var ns = {}; " +
"(function() { " +
" /** @param {number} x */ ns.foo = function(x) {}; })();" +
"(function() { ns.foo(true); })();",
"actual parameter 1 of ns.foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testQualifiedNameInference6() throws Exception {
testTypes(
"var ns = {}; " +
"/** @param {number} x */ ns.foo = function(x) {};" +
"(function() { " +
" ns.foo = function(x) {};" +
" ns.foo(true); })();",
"actual parameter 1 of ns.foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testQualifiedNameInference7() throws Exception {
testTypes(
"var ns = {}; " +
"(function() { " +
" /** @constructor \n * @param {number} x */ " +
" ns.Foo = function(x) {};" +
" /** @param {ns.Foo} x */ function f(x) {}" +
" f(new ns.Foo(true));" +
"})();",
"actual parameter 1 of ns.Foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testQualifiedNameInference8() throws Exception {
testTypes(
"var ns = {}; " +
"(function() { " +
" /** @constructor \n * @param {number} x */ " +
" ns.Foo = function(x) {};" +
"})();" +
"/** @param {ns.Foo} x */ function f(x) {}" +
"f(new ns.Foo(true));",
"Bad type annotation. Unknown type ns.Foo");
}
public void testQualifiedNameInference9() throws Exception {
testTypes(
"var ns = {}; " +
"ns.ns2 = {}; " +
"(function() { " +
" /** @constructor \n * @param {number} x */ " +
" ns.ns2.Foo = function(x) {};" +
" /** @param {ns.ns2.Foo} x */ function f(x) {}" +
" f(new ns.ns2.Foo(true));" +
"})();",
"actual parameter 1 of ns.ns2.Foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testQualifiedNameInference10() throws Exception {
testTypes(
"var ns = {}; " +
"ns.ns2 = {}; " +
"(function() { " +
" /** @interface */ " +
" ns.ns2.Foo = function() {};" +
" /** @constructor \n * @implements {ns.ns2.Foo} */ " +
" function F() {}" +
" (new F());" +
"})();");
}
public void testQualifiedNameInference11() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"function f() {" +
" var x = new Foo();" +
" x.onload = function() {" +
" x.onload = null;" +
" };" +
"}");
}
public void testQualifiedNameInference12() throws Exception {
// We should be able to tell that the two 'this' properties
// are different.
testTypes(
"/** @param {function(this:Object)} x */ function f(x) {}" +
"/** @constructor */ function Foo() {" +
" /** @type {number} */ this.bar = 3;" +
" f(function() { this.bar = true; });" +
"}");
}
public void testSheqRefinedScope() throws Exception {
Node n = parseAndTypeCheck(
"/** @constructor */function A() {}\n" +
"/** @constructor \n @extends A */ function B() {}\n" +
"/** @return {number} */\n" +
"B.prototype.p = function() { return 1; }\n" +
"/** @param {A} a\n @param {B} b */\n" +
"function f(a, b) {\n" +
" b.p();\n" +
" if (a === b) {\n" +
" b.p();\n" +
" }\n" +
"}");
Node nodeC = n.getLastChild().getLastChild().getLastChild().getLastChild()
.getLastChild().getLastChild();
JSType typeC = nodeC.getJSType();
assertTrue(typeC.isNumber());
Node nodeB = nodeC.getFirstChild().getFirstChild();
JSType typeB = nodeB.getJSType();
assertEquals("B", typeB.toString());
}
public void testAssignToUntypedVariable() throws Exception {
Node n = parseAndTypeCheck("var z; z = 1;");
Node assign = n.getLastChild().getFirstChild();
Node node = assign.getFirstChild();
assertFalse(node.getJSType().isUnknownType());
assertEquals("number", node.getJSType().toString());
}
public void testAssignToUntypedProperty() throws Exception {
Node n = parseAndTypeCheck(
"/** @constructor */ function Foo() {}\n" +
"Foo.prototype.a = 1;" +
"(new Foo).a;");
Node node = n.getLastChild().getFirstChild();
assertFalse(node.getJSType().isUnknownType());
assertTrue(node.getJSType().isNumber());
}
public void testNew1() throws Exception {
testTypes("new 4", TypeCheck.NOT_A_CONSTRUCTOR);
}
public void testNew2() throws Exception {
testTypes("var Math = {}; new Math()", TypeCheck.NOT_A_CONSTRUCTOR);
}
public void testNew3() throws Exception {
testTypes("new Date()");
}
public void testNew4() throws Exception {
testTypes("/** @constructor */function A(){}; new A();");
}
public void testNew5() throws Exception {
testTypes("function A(){}; new A();", TypeCheck.NOT_A_CONSTRUCTOR);
}
public void testNew6() throws Exception {
TypeCheckResult p =
parseAndTypeCheckWithScope("/** @constructor */function A(){};" +
"var a = new A();");
JSType aType = p.scope.getVar("a").getType();
assertTrue(aType instanceof ObjectType);
ObjectType aObjectType = (ObjectType) aType;
assertEquals("A", aObjectType.getConstructor().getReferenceName());
}
public void testNew7() throws Exception {
testTypes("/** @param {Function} opt_constructor */" +
"function foo(opt_constructor) {" +
"if (opt_constructor) { new opt_constructor; }" +
"}");
}
public void testNew8() throws Exception {
testTypes("/** @param {Function} opt_constructor */" +
"function foo(opt_constructor) {" +
"new opt_constructor;" +
"}");
}
public void testNew9() throws Exception {
testTypes("/** @param {Function} opt_constructor */" +
"function foo(opt_constructor) {" +
"new (opt_constructor || Array);" +
"}");
}
public void testNew10() throws Exception {
testTypes("var goog = {};" +
"/** @param {Function} opt_constructor */" +
"goog.Foo = function (opt_constructor) {" +
"new (opt_constructor || Array);" +
"}");
}
public void testNew11() throws Exception {
testTypes("/** @param {Function} c1 */" +
"function f(c1) {" +
" var c2 = function(){};" +
" c1.prototype = new c2;" +
"}", TypeCheck.NOT_A_CONSTRUCTOR);
}
public void testNew12() throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope("var a = new Array();");
Var a = p.scope.getVar("a");
assertEquals(ARRAY_TYPE, a.getType());
}
public void testNew13() throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope(
"/** @constructor */function FooBar(){};" +
"var a = new FooBar();");
Var a = p.scope.getVar("a");
assertTrue(a.getType() instanceof ObjectType);
assertEquals("FooBar", a.getType().toString());
}
public void testNew14() throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope(
"/** @constructor */var FooBar = function(){};" +
"var a = new FooBar();");
Var a = p.scope.getVar("a");
assertTrue(a.getType() instanceof ObjectType);
assertEquals("FooBar", a.getType().toString());
}
public void testNew15() throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope(
"var goog = {};" +
"/** @constructor */goog.A = function(){};" +
"var a = new goog.A();");
Var a = p.scope.getVar("a");
assertTrue(a.getType() instanceof ObjectType);
assertEquals("goog.A", a.getType().toString());
}
public void testNew16() throws Exception {
testTypes(
"/** \n" +
" * @param {string} x \n" +
" * @constructor \n" +
" */" +
"function Foo(x) {}" +
"function g() { new Foo(1); }",
"actual parameter 1 of Foo does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testNew17() throws Exception {
testTypes("var goog = {}; goog.x = 3; new goog.x",
"cannot instantiate non-constructor");
}
public void testNew18() throws Exception {
testTypes("var goog = {};" +
"/** @constructor */ goog.F = function() {};" +
"/** @constructor */ goog.G = goog.F;");
}
public void testName1() throws Exception {
assertEquals(VOID_TYPE, testNameNode("undefined"));
}
public void testName2() throws Exception {
assertEquals(OBJECT_FUNCTION_TYPE, testNameNode("Object"));
}
public void testName3() throws Exception {
assertEquals(ARRAY_FUNCTION_TYPE, testNameNode("Array"));
}
public void testName4() throws Exception {
assertEquals(DATE_FUNCTION_TYPE, testNameNode("Date"));
}
public void testName5() throws Exception {
assertEquals(REGEXP_FUNCTION_TYPE, testNameNode("RegExp"));
}
/**
* Type checks a NAME node and retrieve its type.
*/
private JSType testNameNode(String name) {
Node node = Node.newString(Token.NAME, name);
Node parent = new Node(Token.SCRIPT, node);
parent.setInputId(new InputId("code"));
Node externs = new Node(Token.SCRIPT);
externs.setInputId(new InputId("externs"));
Node externAndJsRoot = new Node(Token.BLOCK, externs, parent);
externAndJsRoot.setIsSyntheticBlock(true);
makeTypeCheck().processForTesting(null, parent);
return node.getJSType();
}
public void testBitOperation1() throws Exception {
testTypes("/**@return {void}*/function foo(){ ~foo(); }",
"operator ~ cannot be applied to undefined");
}
public void testBitOperation2() throws Exception {
testTypes("/**@return {void}*/function foo(){var a = foo()<<3;}",
"operator << cannot be applied to undefined");
}
public void testBitOperation3() throws Exception {
testTypes("/**@return {void}*/function foo(){var a = 3<<foo();}",
"operator << cannot be applied to undefined");
}
public void testBitOperation4() throws Exception {
testTypes("/**@return {void}*/function foo(){var a = foo()>>>3;}",
"operator >>> cannot be applied to undefined");
}
public void testBitOperation5() throws Exception {
testTypes("/**@return {void}*/function foo(){var a = 3>>>foo();}",
"operator >>> cannot be applied to undefined");
}
public void testBitOperation6() throws Exception {
testTypes("/**@return {!Object}*/function foo(){var a = foo()&3;}",
"bad left operand to bitwise operator\n" +
"found : Object\n" +
"required: (boolean|null|number|string|undefined)");
}
public void testBitOperation7() throws Exception {
testTypes("var x = null; x |= undefined; x &= 3; x ^= '3'; x |= true;");
}
public void testBitOperation8() throws Exception {
testTypes("var x = void 0; x |= new Number(3);");
}
public void testBitOperation9() throws Exception {
testTypes("var x = void 0; x |= {};",
"bad right operand to bitwise operator\n" +
"found : {}\n" +
"required: (boolean|null|number|string|undefined)");
}
public void testCall1() throws Exception {
testTypes("3();", "number expressions are not callable");
}
public void testCall2() throws Exception {
testTypes("/** @param {!Number} foo*/function bar(foo){ bar('abc'); }",
"actual parameter 1 of bar does not match formal parameter\n" +
"found : string\n" +
"required: Number");
}
public void testCall3() throws Exception {
// We are checking that an unresolved named type can successfully
// meet with a functional type to produce a callable type.
testTypes("/** @type {Function|undefined} */var opt_f;" +
"/** @type {some.unknown.type} */var f1;" +
"var f2 = opt_f || f1;" +
"f2();",
"Bad type annotation. Unknown type some.unknown.type");
}
public void testCall4() throws Exception {
testTypes("/**@param {!RegExp} a*/var foo = function bar(a){ bar('abc'); }",
"actual parameter 1 of bar does not match formal parameter\n" +
"found : string\n" +
"required: RegExp");
}
public void testCall5() throws Exception {
testTypes("/**@param {!RegExp} a*/var foo = function bar(a){ foo('abc'); }",
"actual parameter 1 of foo does not match formal parameter\n" +
"found : string\n" +
"required: RegExp");
}
public void testCall6() throws Exception {
testTypes("/** @param {!Number} foo*/function bar(foo){}" +
"bar('abc');",
"actual parameter 1 of bar does not match formal parameter\n" +
"found : string\n" +
"required: Number");
}
public void testCall7() throws Exception {
testTypes("/** @param {!RegExp} a*/var foo = function bar(a){};" +
"foo('abc');",
"actual parameter 1 of foo does not match formal parameter\n" +
"found : string\n" +
"required: RegExp");
}
public void testCall8() throws Exception {
testTypes("/** @type {Function|number} */var f;f();",
"(Function|number) expressions are " +
"not callable");
}
public void testCall9() throws Exception {
testTypes(
"var goog = {};" +
"/** @constructor */ goog.Foo = function() {};" +
"/** @param {!goog.Foo} a */ var bar = function(a){};" +
"bar('abc');",
"actual parameter 1 of bar does not match formal parameter\n" +
"found : string\n" +
"required: goog.Foo");
}
public void testCall10() throws Exception {
testTypes("/** @type {Function} */var f;f();");
}
public void testCall11() throws Exception {
testTypes("var f = new Function(); f();");
}
public void testFunctionCall1() throws Exception {
testTypes(
"/** @param {number} x */ var foo = function(x) {};" +
"foo.call(null, 3);");
}
public void testFunctionCall2() throws Exception {
testTypes(
"/** @param {number} x */ var foo = function(x) {};" +
"foo.call(null, 'bar');",
"actual parameter 2 of foo.call does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testFunctionCall3() throws Exception {
testTypes(
"/** @param {number} x \n * @constructor */ " +
"var Foo = function(x) { this.bar.call(null, x); };" +
"/** @type {function(number)} */ Foo.prototype.bar;");
}
public void testFunctionCall4() throws Exception {
testTypes(
"/** @param {string} x \n * @constructor */ " +
"var Foo = function(x) { this.bar.call(null, x); };" +
"/** @type {function(number)} */ Foo.prototype.bar;",
"actual parameter 2 of this.bar.call " +
"does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testFunctionCall5() throws Exception {
testTypes(
"/** @param {Function} handler \n * @constructor */ " +
"var Foo = function(handler) { handler.call(this, x); };");
}
public void testFunctionCall6() throws Exception {
testTypes(
"/** @param {Function} handler \n * @constructor */ " +
"var Foo = function(handler) { handler.apply(this, x); };");
}
public void testFunctionCall7() throws Exception {
testTypes(
"/** @param {Function} handler \n * @param {Object} opt_context */ " +
"var Foo = function(handler, opt_context) { " +
" handler.call(opt_context, x);" +
"};");
}
public void testFunctionCall8() throws Exception {
testTypes(
"/** @param {Function} handler \n * @param {Object} opt_context */ " +
"var Foo = function(handler, opt_context) { " +
" handler.apply(opt_context, x);" +
"};");
}
public void testFunctionBind1() throws Exception {
testTypes(
"/** @type {function(string, number): boolean} */" +
"function f(x, y) { return true; }" +
"f.bind(null, 3);",
"actual parameter 2 of f.bind does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testFunctionBind2() throws Exception {
testTypes(
"/** @type {function(number): boolean} */" +
"function f(x) { return true; }" +
"f(f.bind(null, 3)());",
"actual parameter 1 of f does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testFunctionBind3() throws Exception {
testTypes(
"/** @type {function(number, string): boolean} */" +
"function f(x, y) { return true; }" +
"f.bind(null, 3)(true);",
"actual parameter 1 of function does not match formal parameter\n" +
"found : boolean\n" +
"required: string");
}
public void testFunctionBind4() throws Exception {
testTypes(
"/** @param {...number} x */" +
"function f(x) {}" +
"f.bind(null, 3, 3, 3)(true);",
"actual parameter 1 of function does not match formal parameter\n" +
"found : boolean\n" +
"required: (number|undefined)");
}
public void testFunctionBind5() throws Exception {
testTypes(
"/** @param {...number} x */" +
"function f(x) {}" +
"f.bind(null, true)(3, 3, 3);",
"actual parameter 2 of f.bind does not match formal parameter\n" +
"found : boolean\n" +
"required: (number|undefined)");
}
public void testGoogBind1() throws Exception {
// We currently do not support goog.bind natively.
testClosureTypes(
"var goog = {}; goog.bind = function(var_args) {};" +
"/** @type {function(number): boolean} */" +
"function f(x, y) { return true; }" +
"f(goog.bind(f, null, 'x')());",
null);
}
public void testCast2() throws Exception {
// can upcast to a base type.
testTypes("/** @constructor */function base() {}\n" +
"/** @constructor\n @extends {base} */function derived() {}\n" +
"/** @type {base} */ var baz = new derived();\n");
}
public void testCast3() throws Exception {
// cannot downcast
testTypes("/** @constructor */function base() {}\n" +
"/** @constructor @extends {base} */function derived() {}\n" +
"/** @type {!derived} */ var baz = new base();\n",
"initializing variable\n" +
"found : base\n" +
"required: derived");
}
public void testCast4() throws Exception {
// downcast must be explicit
testTypes("/** @constructor */function base() {}\n" +
"/** @constructor\n * @extends {base} */function derived() {}\n" +
"/** @type {!derived} */ var baz = " +
"/** @type {!derived} */(new base());\n");
}
public void testCast5() throws Exception {
// cannot explicitly cast to an unrelated type
testTypes("/** @constructor */function foo() {}\n" +
"/** @constructor */function bar() {}\n" +
"var baz = /** @type {!foo} */(new bar);\n",
"invalid cast - must be a subtype or supertype\n" +
"from: bar\n" +
"to : foo");
}
public void testCast6() throws Exception {
// can explicitly cast to a subtype or supertype
testTypes("/** @constructor */function foo() {}\n" +
"/** @constructor \n @extends foo */function bar() {}\n" +
"var baz = /** @type {!bar} */(new bar);\n" +
"var baz = /** @type {!foo} */(new foo);\n" +
"var baz = /** @type {bar} */(new bar);\n" +
"var baz = /** @type {foo} */(new foo);\n" +
"var baz = /** @type {!foo} */(new bar);\n" +
"var baz = /** @type {!bar} */(new foo);\n" +
"var baz = /** @type {foo} */(new bar);\n" +
"var baz = /** @type {bar} */(new foo);\n");
}
public void testCast7() throws Exception {
testTypes("var x = /** @type {foo} */ (new Object());",
"Bad type annotation. Unknown type foo");
}
public void testCast8() throws Exception {
testTypes("function f() { return /** @type {foo} */ (new Object()); }",
"Bad type annotation. Unknown type foo");
}
public void testCast9() throws Exception {
testTypes("var foo = {};" +
"function f() { return /** @type {foo} */ (new Object()); }",
"Bad type annotation. Unknown type foo");
}
public void testCast10() throws Exception {
testTypes("var foo = function() {};" +
"function f() { return /** @type {foo} */ (new Object()); }",
"Bad type annotation. Unknown type foo");
}
public void testCast11() throws Exception {
testTypes("var goog = {}; goog.foo = {};" +
"function f() { return /** @type {goog.foo} */ (new Object()); }",
"Bad type annotation. Unknown type goog.foo");
}
public void testCast12() throws Exception {
testTypes("var goog = {}; goog.foo = function() {};" +
"function f() { return /** @type {goog.foo} */ (new Object()); }",
"Bad type annotation. Unknown type goog.foo");
}
public void testCast13() throws Exception {
// Test to make sure that the forward-declaration still allows for
// a warning.
testClosureTypes("var goog = {}; " +
"goog.addDependency('zzz.js', ['goog.foo'], []);" +
"goog.foo = function() {};" +
"function f() { return /** @type {goog.foo} */ (new Object()); }",
"Bad type annotation. Unknown type goog.foo");
}
public void testCast14() throws Exception {
// Test to make sure that the forward-declaration still prevents
// some warnings.
testClosureTypes("var goog = {}; " +
"goog.addDependency('zzz.js', ['goog.bar'], []);" +
"function f() { return /** @type {goog.bar} */ (new Object()); }",
null);
}
public void testCast15() throws Exception {
// This fixes a bug where a type cast on an object literal
// would cause a runtime cast exception if the node was visited
// more than once.
//
// Some code assumes that an object literal must have a object type,
// while because of the cast, it could have any type (including
// a union).
testTypes(
"for (var i = 0; i < 10; i++) {" +
"var x = /** @type {Object|number} */ ({foo: 3});" +
"/** @param {number} x */ function f(x) {}" +
"f(x.foo);" +
"f([].foo);" +
"}",
"Property foo never defined on Array");
}
public void testCast16() throws Exception {
// A type cast should not invalidate the checks on the members
testTypes(
"for (var i = 0; i < 10; i++) {" +
"var x = /** @type {Object|number} */ (" +
" {/** @type {string} */ foo: 3});" +
"}",
"assignment to property foo of Object\n" +
"found : number\n" +
"required: string");
}
public void testCast17() throws Exception {
// Mostly verifying that rhino actually understands these JsDocs.
testTypes("/** @constructor */ function Foo() {} \n" +
"/** @type {Foo} */ var x = /** @type {Foo} */ ({})");
testTypes("/** @constructor */ function Foo() {} \n" +
"/** @type {Foo} */ var x = (/** @type {Foo} */ {})");
// Not really encourage because of possible ambiguity but it works.
testTypes("/** @constructor */ function Foo() {} \n" +
"/** @type {Foo} */ var x = /** @type {Foo} */ {}");
}
public void testNestedCasts() throws Exception {
testTypes("/** @constructor */var T = function() {};\n" +
"/** @constructor */var V = function() {};\n" +
"/**\n" +
"* @param {boolean} b\n" +
"* @return {T|V}\n" +
"*/\n" +
"function f(b) { return b ? new T() : new V(); }\n" +
"/**\n" +
"* @param {boolean} b\n" +
"* @return {boolean|undefined}\n" +
"*/\n" +
"function g(b) { return b ? true : undefined; }\n" +
"/** @return {T} */\n" +
"function h() {\n" +
"return /** @type {T} */ (f(/** @type {boolean} */ (g(true))));\n" +
"}");
}
public void testNativeCast1() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"f(String(true));",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testNativeCast2() throws Exception {
testTypes(
"/** @param {string} x */ function f(x) {}" +
"f(Number(true));",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testNativeCast3() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"f(Boolean(''));",
"actual parameter 1 of f does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testNativeCast4() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"f(Error(''));",
"actual parameter 1 of f does not match formal parameter\n" +
"found : Error\n" +
"required: number");
}
public void testBadConstructorCall() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo();",
"Constructor function (new:Foo): undefined should be called " +
"with the \"new\" keyword");
}
public void testTypeof() throws Exception {
testTypes("/**@return {void}*/function foo(){ var a = typeof foo(); }");
}
public void testConstructorType1() throws Exception {
testTypes("/**@constructor*/function Foo(){}" +
"/**@type{!Foo}*/var f = new Date();",
"initializing variable\n" +
"found : Date\n" +
"required: Foo");
}
public void testConstructorType2() throws Exception {
testTypes("/**@constructor*/function Foo(){\n" +
"/**@type{Number}*/this.bar = new Number(5);\n" +
"}\n" +
"/**@type{Foo}*/var f = new Foo();\n" +
"/**@type{Number}*/var n = f.bar;");
}
public void testConstructorType3() throws Exception {
// Reverse the declaration order so that we know that Foo is getting set
// even on an out-of-order declaration sequence.
testTypes("/**@type{Foo}*/var f = new Foo();\n" +
"/**@type{Number}*/var n = f.bar;" +
"/**@constructor*/function Foo(){\n" +
"/**@type{Number}*/this.bar = new Number(5);\n" +
"}\n");
}
public void testConstructorType4() throws Exception {
testTypes("/**@constructor*/function Foo(){\n" +
"/**@type{!Number}*/this.bar = new Number(5);\n" +
"}\n" +
"/**@type{!Foo}*/var f = new Foo();\n" +
"/**@type{!String}*/var n = f.bar;",
"initializing variable\n" +
"found : Number\n" +
"required: String");
}
public void testConstructorType5() throws Exception {
testTypes("/**@constructor*/function Foo(){}\n" +
"if (Foo){}\n");
}
public void testConstructorType6() throws Exception {
testTypes("/** @constructor */\n" +
"function bar() {}\n" +
"function _foo() {\n" +
" /** @param {bar} x */\n" +
" function f(x) {}\n" +
"}");
}
public void testConstructorType7() throws Exception {
TypeCheckResult p =
parseAndTypeCheckWithScope("/** @constructor */function A(){};");
JSType type = p.scope.getVar("A").getType();
assertTrue(type instanceof FunctionType);
FunctionType fType = (FunctionType) type;
assertEquals("A", fType.getReferenceName());
}
public void testConstructorType8() throws Exception {
testTypes(
"var ns = {};" +
"ns.create = function() { return function() {}; };" +
"/** @constructor */ ns.Foo = ns.create();" +
"ns.Foo.prototype = {x: 0, y: 0};" +
"/**\n" +
" * @param {ns.Foo} foo\n" +
" * @return {string}\n" +
" */\n" +
"function f(foo) {" +
" return foo.x;" +
"}",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testConstructorType9() throws Exception {
testTypes(
"var ns = {};" +
"ns.create = function() { return function() {}; };" +
"ns.extend = function(x) { return x; };" +
"/** @constructor */ ns.Foo = ns.create();" +
"ns.Foo.prototype = ns.extend({x: 0, y: 0});" +
"/**\n" +
" * @param {ns.Foo} foo\n" +
" * @return {string}\n" +
" */\n" +
"function f(foo) {" +
" return foo.x;" +
"}");
}
public void testAnonymousPrototype1() throws Exception {
testTypes(
"var ns = {};" +
"/** @constructor */ ns.Foo = function() {" +
" this.bar(3, 5);" +
"};" +
"ns.Foo.prototype = {" +
" bar: function(x) {}" +
"};",
"Function ns.Foo.prototype.bar: called with 2 argument(s). " +
"Function requires at least 1 argument(s) and no more " +
"than 1 argument(s).");
}
public void testAnonymousPrototype2() throws Exception {
testTypes(
"/** @interface */ var Foo = function() {};" +
"Foo.prototype = {" +
" foo: function(x) {}" +
"};" +
"/**\n" +
" * @constructor\n" +
" * @implements {Foo}\n" +
" */ var Bar = function() {};",
"property foo on interface Foo is not implemented by type Bar");
}
public void testAnonymousType1() throws Exception {
testTypes("function f() {}" +
"/** @constructor */\n" +
"f().bar = function() {};");
}
public void testAnonymousType2() throws Exception {
testTypes("function f() {}" +
"/** @interface */\n" +
"f().bar = function() {};");
}
public void testAnonymousType3() throws Exception {
testTypes("function f() {}" +
"/** @enum */\n" +
"f().bar = {FOO: 1};");
}
public void testBang1() throws Exception {
testTypes("/** @param {Object} x\n@return {!Object} */\n" +
"function f(x) { return x; }",
"inconsistent return type\n" +
"found : (Object|null)\n" +
"required: Object");
}
public void testBang2() throws Exception {
testTypes("/** @param {Object} x\n@return {!Object} */\n" +
"function f(x) { return x ? x : new Object(); }");
}
public void testBang3() throws Exception {
testTypes("/** @param {Object} x\n@return {!Object} */\n" +
"function f(x) { return /** @type {!Object} */ (x); }");
}
public void testBang4() throws Exception {
testTypes("/**@param {Object} x\n@param {Object} y\n@return {boolean}*/\n" +
"function f(x, y) {\n" +
"if (typeof x != 'undefined') { return x == y; }\n" +
"else { return x != y; }\n}");
}
public void testBang5() throws Exception {
testTypes("/**@param {Object} x\n@param {Object} y\n@return {boolean}*/\n" +
"function f(x, y) { return !!x && x == y; }");
}
public void testBang6() throws Exception {
testTypes("/** @param {Object?} x\n@return {Object} */\n" +
"function f(x) { return x; }");
}
public void testBang7() throws Exception {
testTypes("/**@param {(Object,string,null)} x\n" +
"@return {(Object,string)}*/function f(x) { return x; }");
}
public void testDefinePropertyOnNullableObject1() throws Exception {
testTypes("/** @type {Object} */ var n = {};\n" +
"/** @type {number} */ n.x = 1;\n" +
"/** @return {boolean} */function f() { return n.x; }",
"inconsistent return type\n" +
"found : number\n" +
"required: boolean");
}
public void testDefinePropertyOnNullableObject2() throws Exception {
testTypes("/** @constructor */ var T = function() {};\n" +
"/** @param {T} t\n@return {boolean} */function f(t) {\n" +
"t.x = 1; return t.x; }",
"inconsistent return type\n" +
"found : number\n" +
"required: boolean");
}
public void testUnknownConstructorInstanceType1() throws Exception {
testTypes("/** @return {Array} */ function g(f) { return new f(); }");
}
public void testUnknownConstructorInstanceType2() throws Exception {
testTypes("function g(f) { return /** @type Array */ new f(); }");
}
public void testUnknownConstructorInstanceType3() throws Exception {
testTypes("function g(f) { var x = new f(); x.a = 1; return x; }");
}
public void testUnknownPrototypeChain() throws Exception {
testTypes("/**\n" +
"* @param {Object} co\n" +
" * @return {Object}\n" +
" */\n" +
"function inst(co) {\n" +
" /** @constructor */\n" +
" var c = function() {};\n" +
" c.prototype = co.prototype;\n" +
" return new c;\n" +
"}");
}
public void testNamespacedConstructor() throws Exception {
Node root = parseAndTypeCheck(
"var goog = {};" +
"/** @constructor */ goog.MyClass = function() {};" +
"/** @return {!goog.MyClass} */ " +
"function foo() { return new goog.MyClass(); }");
JSType typeOfFoo = root.getLastChild().getJSType();
assert(typeOfFoo instanceof FunctionType);
JSType retType = ((FunctionType) typeOfFoo).getReturnType();
assert(retType instanceof ObjectType);
assertEquals("goog.MyClass", ((ObjectType) retType).getReferenceName());
}
public void testComplexNamespace() throws Exception {
String js =
"var goog = {};" +
"goog.foo = {};" +
"goog.foo.bar = 5;";
TypeCheckResult p = parseAndTypeCheckWithScope(js);
// goog type in the scope
JSType googScopeType = p.scope.getVar("goog").getType();
assertTrue(googScopeType instanceof ObjectType);
assertTrue("foo property not present on goog type",
((ObjectType) googScopeType).hasProperty("foo"));
assertFalse("bar property present on goog type",
((ObjectType) googScopeType).hasProperty("bar"));
// goog type on the VAR node
Node varNode = p.root.getFirstChild();
assertEquals(Token.VAR, varNode.getType());
JSType googNodeType = varNode.getFirstChild().getJSType();
assertTrue(googNodeType instanceof ObjectType);
// goog scope type and goog type on VAR node must be the same
assertTrue(googScopeType == googNodeType);
// goog type on the left of the GETPROP node (under fist ASSIGN)
Node getpropFoo1 = varNode.getNext().getFirstChild().getFirstChild();
assertEquals(Token.GETPROP, getpropFoo1.getType());
assertEquals("goog", getpropFoo1.getFirstChild().getString());
JSType googGetpropFoo1Type = getpropFoo1.getFirstChild().getJSType();
assertTrue(googGetpropFoo1Type instanceof ObjectType);
// still the same type as the one on the variable
assertTrue(googGetpropFoo1Type == googScopeType);
// the foo property should be defined on goog
JSType googFooType = ((ObjectType) googScopeType).getPropertyType("foo");
assertTrue(googFooType instanceof ObjectType);
// goog type on the left of the GETPROP lower level node
// (under second ASSIGN)
Node getpropFoo2 = varNode.getNext().getNext()
.getFirstChild().getFirstChild().getFirstChild();
assertEquals(Token.GETPROP, getpropFoo2.getType());
assertEquals("goog", getpropFoo2.getFirstChild().getString());
JSType googGetpropFoo2Type = getpropFoo2.getFirstChild().getJSType();
assertTrue(googGetpropFoo2Type instanceof ObjectType);
// still the same type as the one on the variable
assertTrue(googGetpropFoo2Type == googScopeType);
// goog.foo type on the left of the top level GETPROP node
// (under second ASSIGN)
JSType googFooGetprop2Type = getpropFoo2.getJSType();
assertTrue("goog.foo incorrectly annotated in goog.foo.bar selection",
googFooGetprop2Type instanceof ObjectType);
ObjectType googFooGetprop2ObjectType = (ObjectType) googFooGetprop2Type;
assertFalse("foo property present on goog.foo type",
googFooGetprop2ObjectType.hasProperty("foo"));
assertTrue("bar property not present on goog.foo type",
googFooGetprop2ObjectType.hasProperty("bar"));
assertEquals("bar property on goog.foo type incorrectly inferred",
NUMBER_TYPE, googFooGetprop2ObjectType.getPropertyType("bar"));
}
public void testAddingMethodsUsingPrototypeIdiomSimpleNamespace()
throws Exception {
Node js1Node = parseAndTypeCheck(
"/** @constructor */function A() {}" +
"A.prototype.m1 = 5");
ObjectType instanceType = getInstanceType(js1Node);
assertEquals(NATIVE_PROPERTIES_COUNT + 1,
instanceType.getPropertiesCount());
checkObjectType(instanceType, "m1", NUMBER_TYPE);
}
public void testAddingMethodsUsingPrototypeIdiomComplexNamespace1()
throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope(
"var goog = {};" +
"goog.A = /** @constructor */function() {};" +
"/** @type number */goog.A.prototype.m1 = 5");
testAddingMethodsUsingPrototypeIdiomComplexNamespace(p);
}
public void testAddingMethodsUsingPrototypeIdiomComplexNamespace2()
throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope(
"var goog = {};" +
"/** @constructor */goog.A = function() {};" +
"/** @type number */goog.A.prototype.m1 = 5");
testAddingMethodsUsingPrototypeIdiomComplexNamespace(p);
}
private void testAddingMethodsUsingPrototypeIdiomComplexNamespace(
TypeCheckResult p) {
ObjectType goog = (ObjectType) p.scope.getVar("goog").getType();
assertEquals(NATIVE_PROPERTIES_COUNT + 1, goog.getPropertiesCount());
JSType googA = goog.getPropertyType("A");
assertNotNull(googA);
assertTrue(googA instanceof FunctionType);
FunctionType googAFunction = (FunctionType) googA;
ObjectType classA = googAFunction.getInstanceType();
assertEquals(NATIVE_PROPERTIES_COUNT + 1, classA.getPropertiesCount());
checkObjectType(classA, "m1", NUMBER_TYPE);
}
public void testAddingMethodsPrototypeIdiomAndObjectLiteralSimpleNamespace()
throws Exception {
Node js1Node = parseAndTypeCheck(
"/** @constructor */function A() {}" +
"A.prototype = {m1: 5, m2: true}");
ObjectType instanceType = getInstanceType(js1Node);
assertEquals(NATIVE_PROPERTIES_COUNT + 2,
instanceType.getPropertiesCount());
checkObjectType(instanceType, "m1", NUMBER_TYPE);
checkObjectType(instanceType, "m2", BOOLEAN_TYPE);
}
public void testDontAddMethodsIfNoConstructor()
throws Exception {
Node js1Node = parseAndTypeCheck(
"function A() {}" +
"A.prototype = {m1: 5, m2: true}");
JSType functionAType = js1Node.getFirstChild().getJSType();
assertEquals("function (): undefined", functionAType.toString());
assertEquals(UNKNOWN_TYPE,
U2U_FUNCTION_TYPE.getPropertyType("m1"));
assertEquals(UNKNOWN_TYPE,
U2U_FUNCTION_TYPE.getPropertyType("m2"));
}
public void testFunctionAssignement() throws Exception {
testTypes("/**" +
"* @param {string} ph0" +
"* @param {string} ph1" +
"* @return {string}" +
"*/" +
"function MSG_CALENDAR_ACCESS_ERROR(ph0, ph1) {return ''}" +
"/** @type {Function} */" +
"var MSG_CALENDAR_ADD_ERROR = MSG_CALENDAR_ACCESS_ERROR;");
}
public void testAddMethodsPrototypeTwoWays() throws Exception {
Node js1Node = parseAndTypeCheck(
"/** @constructor */function A() {}" +
"A.prototype = {m1: 5, m2: true};" +
"A.prototype.m3 = 'third property!';");
ObjectType instanceType = getInstanceType(js1Node);
assertEquals("A", instanceType.toString());
assertEquals(NATIVE_PROPERTIES_COUNT + 3,
instanceType.getPropertiesCount());
checkObjectType(instanceType, "m1", NUMBER_TYPE);
checkObjectType(instanceType, "m2", BOOLEAN_TYPE);
checkObjectType(instanceType, "m3", STRING_TYPE);
}
public void testPrototypePropertyTypes() throws Exception {
Node js1Node = parseAndTypeCheck(
"/** @constructor */function A() {\n" +
" /** @type string */ this.m1;\n" +
" /** @type Object? */ this.m2 = {};\n" +
" /** @type boolean */ this.m3;\n" +
"}\n" +
"/** @type string */ A.prototype.m4;\n" +
"/** @type number */ A.prototype.m5 = 0;\n" +
"/** @type boolean */ A.prototype.m6;\n");
ObjectType instanceType = getInstanceType(js1Node);
assertEquals(NATIVE_PROPERTIES_COUNT + 6,
instanceType.getPropertiesCount());
checkObjectType(instanceType, "m1", STRING_TYPE);
checkObjectType(instanceType, "m2",
createUnionType(OBJECT_TYPE, NULL_TYPE));
checkObjectType(instanceType, "m3", BOOLEAN_TYPE);
checkObjectType(instanceType, "m4", STRING_TYPE);
checkObjectType(instanceType, "m5", NUMBER_TYPE);
checkObjectType(instanceType, "m6", BOOLEAN_TYPE);
}
public void testValueTypeBuiltInPrototypePropertyType() throws Exception {
Node node = parseAndTypeCheck("\"x\".charAt(0)");
assertEquals(STRING_TYPE, node.getFirstChild().getFirstChild().getJSType());
}
public void testDeclareBuiltInConstructor() throws Exception {
// Built-in prototype properties should be accessible
// even if the built-in constructor is declared.
Node node = parseAndTypeCheck(
"/** @constructor */ var String = function(opt_str) {};\n" +
"(new String(\"x\")).charAt(0)");
assertEquals(STRING_TYPE, node.getLastChild().getFirstChild().getJSType());
}
public void testExtendBuiltInType1() throws Exception {
String externs =
"/** @constructor */ var String = function(opt_str) {};\n" +
"/**\n" +
"* @param {number} start\n" +
"* @param {number} opt_length\n" +
"* @return {string}\n" +
"*/\n" +
"String.prototype.substr = function(start, opt_length) {};\n";
Node n1 = parseAndTypeCheck(externs + "(new String(\"x\")).substr(0,1);");
assertEquals(STRING_TYPE, n1.getLastChild().getFirstChild().getJSType());
}
public void testExtendBuiltInType2() throws Exception {
String externs =
"/** @constructor */ var String = function(opt_str) {};\n" +
"/**\n" +
"* @param {number} start\n" +
"* @param {number} opt_length\n" +
"* @return {string}\n" +
"*/\n" +
"String.prototype.substr = function(start, opt_length) {};\n";
Node n2 = parseAndTypeCheck(externs + "\"x\".substr(0,1);");
assertEquals(STRING_TYPE, n2.getLastChild().getFirstChild().getJSType());
}
public void testExtendFunction1() throws Exception {
Node n = parseAndTypeCheck("/**@return {number}*/Function.prototype.f = " +
"function() { return 1; };\n" +
"(new Function()).f();");
JSType type = n.getLastChild().getLastChild().getJSType();
assertEquals(NUMBER_TYPE, type);
}
public void testExtendFunction2() throws Exception {
Node n = parseAndTypeCheck("/**@return {number}*/Function.prototype.f = " +
"function() { return 1; };\n" +
"(function() {}).f();");
JSType type = n.getLastChild().getLastChild().getJSType();
assertEquals(NUMBER_TYPE, type);
}
public void testInheritanceCheck1() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"Sub.prototype.foo = function() {};");
}
public void testInheritanceCheck2() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override */Sub.prototype.foo = function() {};",
"property foo not defined on any superclass of Sub");
}
public void testInheritanceCheck3() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"Super.prototype.foo = function() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"Sub.prototype.foo = function() {};",
"property foo already defined on superclass Super; " +
"use @override to override it");
}
public void testInheritanceCheck4() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"Super.prototype.foo = function() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override */Sub.prototype.foo = function() {};");
}
public void testInheritanceCheck5() throws Exception {
testTypes(
"/** @constructor */function Root() {};" +
"Root.prototype.foo = function() {};" +
"/** @constructor\n @extends {Root} */function Super() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"Sub.prototype.foo = function() {};",
"property foo already defined on superclass Root; " +
"use @override to override it");
}
public void testInheritanceCheck6() throws Exception {
testTypes(
"/** @constructor */function Root() {};" +
"Root.prototype.foo = function() {};" +
"/** @constructor\n @extends {Root} */function Super() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override */Sub.prototype.foo = function() {};");
}
public void testInheritanceCheck7() throws Exception {
testTypes(
"var goog = {};" +
"/** @constructor */goog.Super = function() {};" +
"goog.Super.prototype.foo = 3;" +
"/** @constructor\n @extends {goog.Super} */goog.Sub = function() {};" +
"goog.Sub.prototype.foo = 5;",
"property foo already defined on superclass goog.Super; " +
"use @override to override it");
}
public void testInheritanceCheck8() throws Exception {
testTypes(
"var goog = {};" +
"/** @constructor */goog.Super = function() {};" +
"goog.Super.prototype.foo = 3;" +
"/** @constructor\n @extends {goog.Super} */goog.Sub = function() {};" +
"/** @override */goog.Sub.prototype.foo = 5;");
}
public void testInheritanceCheck9_1() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"Super.prototype.foo = function() { return 3; };" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override\n @return {number} */Sub.prototype.foo =\n" +
"function() { return 1; };");
}
public void testInheritanceCheck9_2() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"/** @return {number} */" +
"Super.prototype.foo = function() { return 1; };" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override */Sub.prototype.foo =\n" +
"function() {};");
}
public void testInheritanceCheck9_3() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"/** @return {number} */" +
"Super.prototype.foo = function() { return 1; };" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override\n @return {string} */Sub.prototype.foo =\n" +
"function() { return \"some string\" };",
"mismatch of the foo property type and the type of the property it " +
"overrides from superclass Super\n" +
"original: function (this:Super): number\n" +
"override: function (this:Sub): string");
}
public void testInheritanceCheck10_1() throws Exception {
testTypes(
"/** @constructor */function Root() {};" +
"Root.prototype.foo = function() { return 3; };" +
"/** @constructor\n @extends {Root} */function Super() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override\n @return {number} */Sub.prototype.foo =\n" +
"function() { return 1; };");
}
public void testInheritanceCheck10_2() throws Exception {
testTypes(
"/** @constructor */function Root() {};" +
"/** @return {number} */" +
"Root.prototype.foo = function() { return 1; };" +
"/** @constructor\n @extends {Root} */function Super() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override */Sub.prototype.foo =\n" +
"function() {};");
}
public void testInheritanceCheck10_3() throws Exception {
testTypes(
"/** @constructor */function Root() {};" +
"/** @return {number} */" +
"Root.prototype.foo = function() { return 1; };" +
"/** @constructor\n @extends {Root} */function Super() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override\n @return {string} */Sub.prototype.foo =\n" +
"function() { return \"some string\" };",
"mismatch of the foo property type and the type of the property it " +
"overrides from superclass Root\n" +
"original: function (this:Root): number\n" +
"override: function (this:Sub): string");
}
public void testInterfaceInheritanceCheck11() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"/** @param {number} bar */Super.prototype.foo = function(bar) {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override\n @param {string} bar */Sub.prototype.foo =\n" +
"function(bar) {};",
"mismatch of the foo property type and the type of the property it " +
"overrides from superclass Super\n" +
"original: function (this:Super, number): undefined\n" +
"override: function (this:Sub, string): undefined");
}
public void testInheritanceCheck12() throws Exception {
testTypes(
"var goog = {};" +
"/** @constructor */goog.Super = function() {};" +
"goog.Super.prototype.foo = 3;" +
"/** @constructor\n @extends {goog.Super} */goog.Sub = function() {};" +
"/** @override */goog.Sub.prototype.foo = \"some string\";",
"mismatch of the foo property type and the type of the property it " +
"overrides from superclass goog.Super\n" +
"original: number\n" +
"override: string");
}
public void testInheritanceCheck13() throws Exception {
testTypes(
"var goog = {};\n" +
"/** @constructor\n @extends {goog.Missing} */function Sub() {};" +
"/** @override */Sub.prototype.foo = function() {};",
"Bad type annotation. Unknown type goog.Missing");
}
public void testInheritanceCheck14() throws Exception {
testClosureTypes(
"var goog = {};\n" +
"/** @constructor\n @extends {goog.Missing} */\n" +
"goog.Super = function() {};\n" +
"/** @constructor\n @extends {goog.Super} */function Sub() {};" +
"/** @override */Sub.prototype.foo = function() {};",
"Bad type annotation. Unknown type goog.Missing");
}
public void testInheritanceCheck15() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"/** @param {number} bar */Super.prototype.foo;" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override\n @param {number} bar */Sub.prototype.foo =\n" +
"function(bar) {};");
}
public void testInheritanceCheck16() throws Exception {
testTypes(
"var goog = {};" +
"/** @constructor */goog.Super = function() {};" +
"/** @type {number} */ goog.Super.prototype.foo = 3;" +
"/** @constructor\n @extends {goog.Super} */goog.Sub = function() {};" +
"/** @type {number} */ goog.Sub.prototype.foo = 5;",
"property foo already defined on superclass goog.Super; " +
"use @override to override it");
}
public void testInterfacePropertyOverride1() throws Exception {
testTypes(
"/** @interface */function Super() {};" +
"/** @desc description */Super.prototype.foo = function() {};" +
"/** @interface\n @extends {Super} */function Sub() {};" +
"/** @desc description */Sub.prototype.foo = function() {};");
}
public void testInterfacePropertyOverride2() throws Exception {
testTypes(
"/** @interface */function Root() {};" +
"/** @desc description */Root.prototype.foo = function() {};" +
"/** @interface\n @extends {Root} */function Super() {};" +
"/** @interface\n @extends {Super} */function Sub() {};" +
"/** @desc description */Sub.prototype.foo = function() {};");
}
public void testInterfaceInheritanceCheck1() throws Exception {
testTypes(
"/** @interface */function Super() {};" +
"/** @desc description */Super.prototype.foo = function() {};" +
"/** @constructor\n @implements {Super} */function Sub() {};" +
"Sub.prototype.foo = function() {};",
"property foo already defined on interface Super; use @override to " +
"override it");
}
public void testInterfaceInheritanceCheck2() throws Exception {
testTypes(
"/** @interface */function Super() {};" +
"/** @desc description */Super.prototype.foo = function() {};" +
"/** @constructor\n @implements {Super} */function Sub() {};" +
"/** @override */Sub.prototype.foo = function() {};");
}
public void testInterfaceInheritanceCheck3() throws Exception {
testTypes(
"/** @interface */function Root() {};" +
"/** @return {number} */Root.prototype.foo = function() {};" +
"/** @interface\n @extends {Root} */function Super() {};" +
"/** @constructor\n @implements {Super} */function Sub() {};" +
"/** @return {number} */Sub.prototype.foo = function() { return 1;};",
"property foo already defined on interface Root; use @override to " +
"override it");
}
public void testInterfaceInheritanceCheck4() throws Exception {
testTypes(
"/** @interface */function Root() {};" +
"/** @return {number} */Root.prototype.foo = function() {};" +
"/** @interface\n @extends {Root} */function Super() {};" +
"/** @constructor\n @implements {Super} */function Sub() {};" +
"/** @override\n * @return {number} */Sub.prototype.foo =\n" +
"function() { return 1;};");
}
public void testInterfaceInheritanceCheck5() throws Exception {
testTypes(
"/** @interface */function Super() {};" +
"/** @return {string} */Super.prototype.foo = function() {};" +
"/** @constructor\n @implements {Super} */function Sub() {};" +
"/** @override\n @return {number} */Sub.prototype.foo =\n" +
"function() { return 1; };",
"mismatch of the foo property type and the type of the property it " +
"overrides from interface Super\n" +
"original: function (this:Super): string\n" +
"override: function (this:Sub): number");
}
public void testInterfaceInheritanceCheck6() throws Exception {
testTypes(
"/** @interface */function Root() {};" +
"/** @return {string} */Root.prototype.foo = function() {};" +
"/** @interface\n @extends {Root} */function Super() {};" +
"/** @constructor\n @implements {Super} */function Sub() {};" +
"/** @override\n @return {number} */Sub.prototype.foo =\n" +
"function() { return 1; };",
"mismatch of the foo property type and the type of the property it " +
"overrides from interface Root\n" +
"original: function (this:Root): string\n" +
"override: function (this:Sub): number");
}
public void testInterfaceInheritanceCheck7() throws Exception {
testTypes(
"/** @interface */function Super() {};" +
"/** @param {number} bar */Super.prototype.foo = function(bar) {};" +
"/** @constructor\n @implements {Super} */function Sub() {};" +
"/** @override\n @param {string} bar */Sub.prototype.foo =\n" +
"function(bar) {};",
"mismatch of the foo property type and the type of the property it " +
"overrides from interface Super\n" +
"original: function (this:Super, number): undefined\n" +
"override: function (this:Sub, string): undefined");
}
public void testInterfaceInheritanceCheck8() throws Exception {
testTypes(
"/** @constructor\n @implements {Super} */function Sub() {};" +
"/** @override */Sub.prototype.foo = function() {};",
new String[] {
"Bad type annotation. Unknown type Super",
"property foo not defined on any superclass of Sub"
});
}
public void testInterfaceInheritanceCheck9() throws Exception {
testTypes(
"/** @interface */ function I() {}" +
"/** @return {number} */ I.prototype.bar = function() {};" +
"/** @constructor */ function F() {}" +
"/** @return {number} */ F.prototype.bar = function() {return 3; };" +
"/** @return {number} */ F.prototype.foo = function() {return 3; };" +
"/** @constructor \n * @extends {F} \n * @implements {I} */ " +
"function G() {}" +
"/** @return {string} */ function f() { return new G().bar(); }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testInterfaceInheritanceCheck10() throws Exception {
testTypes(
"/** @interface */ function I() {}" +
"/** @return {number} */ I.prototype.bar = function() {};" +
"/** @constructor */ function F() {}" +
"/** @return {number} */ F.prototype.foo = function() {return 3; };" +
"/** @constructor \n * @extends {F} \n * @implements {I} */ " +
"function G() {}" +
"/** @return {number} \n * @override */ " +
"G.prototype.bar = G.prototype.foo;" +
"/** @return {string} */ function f() { return new G().bar(); }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testInterfaceInheritanceCheck12() throws Exception {
testTypes(
"/** @interface */ function I() {};\n" +
"/** @type {string} */ I.prototype.foobar;\n" +
"/** \n * @constructor \n * @implements {I} */\n" +
"function C() {\n" +
"/** \n * @type {number} */ this.foobar = 2;};\n" +
"/** @type {I} */ \n var test = new C(); alert(test.foobar);",
"mismatch of the foobar property type and the type of the property" +
" it overrides from interface I\n" +
"original: string\n" +
"override: number");
}
public void testInterfaceInheritanceCheck13() throws Exception {
testTypes(
"function abstractMethod() {};\n" +
"/** @interface */var base = function() {};\n" +
"/** @extends {base} \n @interface */ var Int = function() {}\n" +
"/** @type {{bar : !Function}} */ var x; \n" +
"/** @type {!Function} */ base.prototype.bar = abstractMethod; \n" +
"/** @type {Int} */ foo;\n" +
"foo.bar();");
}
public void testInterfacePropertyNotImplemented() throws Exception {
testTypes(
"/** @interface */function Int() {};" +
"/** @desc description */Int.prototype.foo = function() {};" +
"/** @constructor\n @implements {Int} */function Foo() {};",
"property foo on interface Int is not implemented by type Foo");
}
public void testInterfacePropertyNotImplemented2() throws Exception {
testTypes(
"/** @interface */function Int() {};" +
"/** @desc description */Int.prototype.foo = function() {};" +
"/** @interface \n @extends {Int} */function Int2() {};" +
"/** @constructor\n @implements {Int2} */function Foo() {};",
"property foo on interface Int is not implemented by type Foo");
}
public void testStubConstructorImplementingInterface() throws Exception {
// This does not throw a warning for unimplemented property because Foo is
// just a stub.
testTypes(
// externs
"/** @interface */ function Int() {}\n" +
"/** @desc description */Int.prototype.foo = function() {};" +
"/** @constructor \n @implements {Int} */ var Foo;\n",
"", null, false);
}
public void testObjectLiteral() throws Exception {
Node n = parseAndTypeCheck("var a = {m1: 7, m2: 'hello'}");
Node nameNode = n.getFirstChild().getFirstChild();
Node objectNode = nameNode.getFirstChild();
// node extraction
assertEquals(Token.NAME, nameNode.getType());
assertEquals(Token.OBJECTLIT, objectNode.getType());
// value's type
ObjectType objectType =
(ObjectType) objectNode.getJSType();
assertEquals(NUMBER_TYPE, objectType.getPropertyType("m1"));
assertEquals(STRING_TYPE, objectType.getPropertyType("m2"));
// variable's type
assertEquals(objectType, nameNode.getJSType());
}
public void testObjectLiteralDeclaration1() throws Exception {
testTypes(
"var x = {" +
"/** @type {boolean} */ abc: true," +
"/** @type {number} */ 'def': 0," +
"/** @type {string} */ 3: 'fgh'" +
"};");
}
public void testObjectLiteralDeclaration2() throws Exception {
testTypes(
"var x = {" +
" /** @type {boolean} */ abc: true" +
"};" +
"x.abc = 0;",
"assignment to property abc of x\n" +
"found : number\n" +
"required: boolean");
}
public void testObjectLiteralDeclaration3() throws Exception {
testTypes(
"/** @param {{foo: !Function}} x */ function f(x) {}" +
"f({foo: function() {}});");
}
public void testObjectLiteralDeclaration4() throws Exception {
testClosureTypes(
"var x = {" +
" /** @param {boolean} x */ abc: function(x) {}" +
"};" +
"/**\n" +
" * @param {string} x\n" +
" * @suppress {duplicate}\n" +
" */ x.abc = function(x) {};",
"assignment to property abc of x\n" +
"found : function (string): undefined\n" +
"required: function (boolean): undefined");
// TODO(user): suppress {duplicate} currently also silence the
// redefining type error in the TypeValidator. May be it needs
// a new suppress name instead?
}
public void testObjectLiteralDeclaration5() throws Exception {
testTypes(
"var x = {" +
" /** @param {boolean} x */ abc: function(x) {}" +
"};" +
"/**\n" +
" * @param {boolean} x\n" +
" * @suppress {duplicate}\n" +
" */ x.abc = function(x) {};");
}
public void testObjectLiteralDeclaration6() throws Exception {
testTypes(
"var x = {};" +
"/**\n" +
" * @param {boolean} x\n" +
" * @suppress {duplicate}\n" +
" */ x.abc = function(x) {};" +
"x = {" +
" /**\n" +
" * @param {boolean} x\n" +
" * @suppress {duplicate}\n" +
" */" +
" abc: function(x) {}" +
"};");
}
public void testObjectLiteralDeclaration7() throws Exception {
testTypes(
"var x = {};" +
"/**\n" +
" * @type {function(boolean): undefined}\n" +
" */ x.abc = function(x) {};" +
"x = {" +
" /**\n" +
" * @param {boolean} x\n" +
" * @suppress {duplicate}\n" +
" */" +
" abc: function(x) {}" +
"};");
}
public void testCallDateConstructorAsFunction() throws Exception {
// ECMA-262 15.9.2: When Date is called as a function rather than as a
// constructor, it returns a string.
Node n = parseAndTypeCheck("Date()");
assertEquals(STRING_TYPE, n.getFirstChild().getFirstChild().getJSType());
}
// According to ECMA-262, Error & Array function calls are equivalent to
// constructor calls.
public void testCallErrorConstructorAsFunction() throws Exception {
Node n = parseAndTypeCheck("Error('x')");
assertEquals(ERROR_TYPE,
n.getFirstChild().getFirstChild().getJSType());
}
public void testCallArrayConstructorAsFunction() throws Exception {
Node n = parseAndTypeCheck("Array()");
assertEquals(ARRAY_TYPE,
n.getFirstChild().getFirstChild().getJSType());
}
public void testPropertyTypeOfUnionType() throws Exception {
testTypes("var a = {};" +
"/** @constructor */ a.N = function() {};\n" +
"a.N.prototype.p = 1;\n" +
"/** @constructor */ a.S = function() {};\n" +
"a.S.prototype.p = 'a';\n" +
"/** @param {!a.N|!a.S} x\n@return {string} */\n" +
"var f = function(x) { return x.p; };",
"inconsistent return type\n" +
"found : (number|string)\n" +
"required: string");
}
// TODO(user): We should flag these as invalid. This will probably happen
// when we make sure the interface is never referenced outside of its
// definition. We might want more specific and helpful error messages.
//public void testWarningOnInterfacePrototype() throws Exception {
// testTypes("/** @interface */ u.T = function() {};\n" +
// "/** @return {number} */ u.T.prototype = function() { };",
// "e of its definition");
//}
//
//public void testBadPropertyOnInterface1() throws Exception {
// testTypes("/** @interface */ u.T = function() {};\n" +
// "/** @return {number} */ u.T.f = function() { return 1;};",
// "cannot reference an interface ouside of its definition");
//}
//
//public void testBadPropertyOnInterface2() throws Exception {
// testTypes("/** @interface */ function T() {};\n" +
// "/** @return {number} */ T.f = function() { return 1;};",
// "cannot reference an interface ouside of its definition");
//}
//
//public void testBadPropertyOnInterface3() throws Exception {
// testTypes("/** @interface */ u.T = function() {}; u.T.x",
// "cannot reference an interface ouside of its definition");
//}
//
//public void testBadPropertyOnInterface4() throws Exception {
// testTypes("/** @interface */ function T() {}; T.x;",
// "cannot reference an interface ouside of its definition");
//}
public void testAnnotatedPropertyOnInterface1() throws Exception {
// For interfaces we must allow function definitions that don't have a
// return statement, even though they declare a returned type.
testTypes("/** @interface */ u.T = function() {};\n" +
"/** @return {number} */ u.T.prototype.f = function() {};");
}
public void testAnnotatedPropertyOnInterface2() throws Exception {
testTypes("/** @interface */ u.T = function() {};\n" +
"/** @return {number} */ u.T.prototype.f = function() { };");
}
public void testAnnotatedPropertyOnInterface3() throws Exception {
testTypes("/** @interface */ function T() {};\n" +
"/** @return {number} */ T.prototype.f = function() { };");
}
public void testAnnotatedPropertyOnInterface4() throws Exception {
testTypes(
CLOSURE_DEFS +
"/** @interface */ function T() {};\n" +
"/** @return {number} */ T.prototype.f = goog.abstractMethod;");
}
// TODO(user): If we want to support this syntax we have to warn about
// missing annotations.
//public void testWarnUnannotatedPropertyOnInterface1() throws Exception {
// testTypes("/** @interface */ u.T = function () {}; u.T.prototype.x;",
// "interface property x is not annotated");
//}
//
//public void testWarnUnannotatedPropertyOnInterface2() throws Exception {
// testTypes("/** @interface */ function T() {}; T.prototype.x;",
// "interface property x is not annotated");
//}
public void testWarnUnannotatedPropertyOnInterface5() throws Exception {
testTypes("/** @interface */ u.T = function () {};\n" +
"/** @desc x does something */u.T.prototype.x = function() {};");
}
public void testWarnUnannotatedPropertyOnInterface6() throws Exception {
testTypes("/** @interface */ function T() {};\n" +
"/** @desc x does something */T.prototype.x = function() {};");
}
// TODO(user): If we want to support this syntax we have to warn about
// the invalid type of the interface member.
//public void testWarnDataPropertyOnInterface1() throws Exception {
// testTypes("/** @interface */ u.T = function () {};\n" +
// "/** @type {number} */u.T.prototype.x;",
// "interface members can only be plain functions");
//}
public void testDataPropertyOnInterface1() throws Exception {
testTypes("/** @interface */ function T() {};\n" +
"/** @type {number} */T.prototype.x;");
}
public void testDataPropertyOnInterface2() throws Exception {
reportMissingOverrides = CheckLevel.OFF;
testTypes("/** @interface */ function T() {};\n" +
"/** @type {number} */T.prototype.x;\n" +
"/** @constructor \n" +
" * @implements {T} \n" +
" */\n" +
"function C() {}\n" +
"C.prototype.x = 'foo';",
"mismatch of the x property type and the type of the property it " +
"overrides from interface T\n" +
"original: number\n" +
"override: string");
}
public void testDataPropertyOnInterface3() throws Exception {
testTypes("/** @interface */ function T() {};\n" +
"/** @type {number} */T.prototype.x;\n" +
"/** @constructor \n" +
" * @implements {T} \n" +
" */\n" +
"function C() {}\n" +
"/** @override */\n" +
"C.prototype.x = 'foo';",
"mismatch of the x property type and the type of the property it " +
"overrides from interface T\n" +
"original: number\n" +
"override: string");
}
public void testDataPropertyOnInterface4() throws Exception {
testTypes("/** @interface */ function T() {};\n" +
"/** @type {number} */T.prototype.x;\n" +
"/** @constructor \n" +
" * @implements {T} \n" +
" */\n" +
"function C() { /** @type {string} */ \n this.x = 'foo'; }\n",
"mismatch of the x property type and the type of the property it " +
"overrides from interface T\n" +
"original: number\n" +
"override: string");
}
public void testWarnDataPropertyOnInterface3() throws Exception {
testTypes("/** @interface */ u.T = function () {};\n" +
"/** @type {number} */u.T.prototype.x = 1;",
"interface members can only be empty property declarations, "
+ "empty functions, or goog.abstractMethod");
}
public void testWarnDataPropertyOnInterface4() throws Exception {
testTypes("/** @interface */ function T() {};\n" +
"/** @type {number} */T.prototype.x = 1;",
"interface members can only be empty property declarations, "
+ "empty functions, or goog.abstractMethod");
}
// TODO(user): If we want to support this syntax we should warn about the
// mismatching types in the two tests below.
//public void testErrorMismatchingPropertyOnInterface1() throws Exception {
// testTypes("/** @interface */ u.T = function () {};\n" +
// "/** @param {Number} foo */u.T.prototype.x =\n" +
// "/** @param {String} foo */function(foo) {};",
// "found : \n" +
// "required: ");
//}
//
//public void testErrorMismatchingPropertyOnInterface2() throws Exception {
// testTypes("/** @interface */ function T() {};\n" +
// "/** @return {number} */T.prototype.x =\n" +
// "/** @return {string} */function() {};",
// "found : \n" +
// "required: ");
//}
// TODO(user): We should warn about this (bar is missing an annotation). We
// probably don't want to warn about all missing parameter annotations, but
// we should be as strict as possible regarding interfaces.
//public void testErrorMismatchingPropertyOnInterface3() throws Exception {
// testTypes("/** @interface */ u.T = function () {};\n" +
// "/** @param {Number} foo */u.T.prototype.x =\n" +
// "function(foo, bar) {};",
// "found : \n" +
// "required: ");
//}
public void testErrorMismatchingPropertyOnInterface4() throws Exception {
testTypes("/** @interface */ u.T = function () {};\n" +
"/** @param {Number} foo */u.T.prototype.x =\n" +
"function() {};",
"parameter foo does not appear in u.T.prototype.x's parameter list");
}
public void testErrorMismatchingPropertyOnInterface5() throws Exception {
testTypes("/** @interface */ function T() {};\n" +
"/** @type {number} */T.prototype.x = function() { };",
"assignment to property x of T.prototype\n" +
"found : function (): undefined\n" +
"required: number");
}
public void testErrorMismatchingPropertyOnInterface6() throws Exception {
testClosureTypesMultipleWarnings(
"/** @interface */ function T() {};\n" +
"/** @return {number} */T.prototype.x = 1",
Lists.newArrayList(
"assignment to property x of T.prototype\n" +
"found : number\n" +
"required: function (this:T): number",
"interface members can only be empty property declarations, " +
"empty functions, or goog.abstractMethod"));
}
public void testInterfaceNonEmptyFunction() throws Exception {
testTypes("/** @interface */ function T() {};\n" +
"T.prototype.x = function() { return 'foo'; }",
"interface member functions must have an empty body"
);
}
public void testDoubleNestedInterface() throws Exception {
testTypes("/** @interface */ var I1 = function() {};\n" +
"/** @interface */ I1.I2 = function() {};\n" +
"/** @interface */ I1.I2.I3 = function() {};\n");
}
public void testStaticDataPropertyOnNestedInterface() throws Exception {
testTypes("/** @interface */ var I1 = function() {};\n" +
"/** @interface */ I1.I2 = function() {};\n" +
"/** @type {number} */ I1.I2.x = 1;\n");
}
public void testInterfaceInstantiation() throws Exception {
testTypes("/** @interface */var f = function(){}; new f",
"cannot instantiate non-constructor");
}
public void testPrototypeLoop() throws Exception {
testClosureTypesMultipleWarnings(
suppressMissingProperty("foo") +
"/** @constructor \n * @extends {T} */var T = function() {};" +
"alert((new T).foo);",
Lists.newArrayList(
"Parse error. Cycle detected in inheritance chain of type T",
"Could not resolve type in @extends tag of T"));
}
public void testDirectPrototypeAssign() throws Exception {
// For now, we just ignore @type annotations on the prototype.
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @constructor */ function Bar() {}" +
"/** @type {Array} */ Bar.prototype = new Foo()");
}
// In all testResolutionViaRegistry* tests, since u is unknown, u.T can only
// be resolved via the registry and not via properties.
public void testResolutionViaRegistry1() throws Exception {
testTypes("/** @constructor */ u.T = function() {};\n" +
"/** @type {(number|string)} */ u.T.prototype.a;\n" +
"/**\n" +
"* @param {u.T} t\n" +
"* @return {string}\n" +
"*/\n" +
"var f = function(t) { return t.a; };",
"inconsistent return type\n" +
"found : (number|string)\n" +
"required: string");
}
public void testResolutionViaRegistry2() throws Exception {
testTypes(
"/** @constructor */ u.T = function() {" +
" this.a = 0; };\n" +
"/**\n" +
"* @param {u.T} t\n" +
"* @return {string}\n" +
"*/\n" +
"var f = function(t) { return t.a; };",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testResolutionViaRegistry3() throws Exception {
testTypes("/** @constructor */ u.T = function() {};\n" +
"/** @type {(number|string)} */ u.T.prototype.a = 0;\n" +
"/**\n" +
"* @param {u.T} t\n" +
"* @return {string}\n" +
"*/\n" +
"var f = function(t) { return t.a; };",
"inconsistent return type\n" +
"found : (number|string)\n" +
"required: string");
}
public void testResolutionViaRegistry4() throws Exception {
testTypes("/** @constructor */ u.A = function() {};\n" +
"/**\n* @constructor\n* @extends {u.A}\n*/\nu.A.A = function() {}\n;" +
"/**\n* @constructor\n* @extends {u.A}\n*/\nu.A.B = function() {};\n" +
"var ab = new u.A.B();\n" +
"/** @type {!u.A} */ var a = ab;\n" +
"/** @type {!u.A.A} */ var aa = ab;\n",
"initializing variable\n" +
"found : u.A.B\n" +
"required: u.A.A");
}
public void testResolutionViaRegistry5() throws Exception {
Node n = parseAndTypeCheck("/** @constructor */ u.T = function() {}; u.T");
JSType type = n.getLastChild().getLastChild().getJSType();
assertFalse(type.isUnknownType());
assertTrue(type instanceof FunctionType);
assertEquals("u.T",
((FunctionType) type).getInstanceType().getReferenceName());
}
public void testGatherProperyWithoutAnnotation1() throws Exception {
Node n = parseAndTypeCheck("/** @constructor */ var T = function() {};" +
"/** @type {!T} */var t; t.x; t;");
JSType type = n.getLastChild().getLastChild().getJSType();
assertFalse(type.isUnknownType());
assertTrue(type instanceof ObjectType);
ObjectType objectType = (ObjectType) type;
assertFalse(objectType.hasProperty("x"));
assertEquals(
Lists.newArrayList(objectType),
registry.getTypesWithProperty("x"));
}
public void testGatherProperyWithoutAnnotation2() throws Exception {
TypeCheckResult ns =
parseAndTypeCheckWithScope("/** @type {!Object} */var t; t.x; t;");
Node n = ns.root;
Scope s = ns.scope;
JSType type = n.getLastChild().getLastChild().getJSType();
assertFalse(type.isUnknownType());
assertEquals(type, OBJECT_TYPE);
assertTrue(type instanceof ObjectType);
ObjectType objectType = (ObjectType) type;
assertFalse(objectType.hasProperty("x"));
assertEquals(
Lists.newArrayList(OBJECT_TYPE),
registry.getTypesWithProperty("x"));
}
public void testFunctionMasksVariableBug() throws Exception {
testTypes("var x = 4; var f = function x(b) { return b ? 1 : x(true); };",
"function x masks variable (IE bug)");
}
public void testDfa1() throws Exception {
testTypes("var x = null;\n x = 1;\n /** @type number */ var y = x;");
}
public void testDfa2() throws Exception {
testTypes("function u() {}\n" +
"/** @return {number} */ function f() {\nvar x = 'todo';\n" +
"if (u()) { x = 1; } else { x = 2; } return x;\n}");
}
public void testDfa3() throws Exception {
testTypes("function u() {}\n" +
"/** @return {number} */ function f() {\n" +
"/** @type {number|string} */ var x = 'todo';\n" +
"if (u()) { x = 1; } else { x = 2; } return x;\n}");
}
public void testDfa4() throws Exception {
testTypes("/** @param {Date?} d */ function f(d) {\n" +
"if (!d) { return; }\n" +
"/** @type {!Date} */ var e = d;\n}");
}
public void testDfa5() throws Exception {
testTypes("/** @return {string?} */ function u() {return 'a';}\n" +
"/** @param {string?} x\n@return {string} */ function f(x) {\n" +
"while (!x) { x = u(); }\nreturn x;\n}");
}
public void testDfa6() throws Exception {
testTypes("/** @return {Object?} */ function u() {return {};}\n" +
"/** @param {Object?} x */ function f(x) {\n" +
"while (x) { x = u(); if (!x) { x = u(); } }\n}");
}
public void testDfa7() throws Exception {
testTypes("/** @constructor */ var T = function() {};\n" +
"/** @type {Date?} */ T.prototype.x = null;\n" +
"/** @param {!T} t */ function f(t) {\n" +
"if (!t.x) { return; }\n" +
"/** @type {!Date} */ var e = t.x;\n}");
}
public void testDfa8() throws Exception {
testTypes("/** @constructor */ var T = function() {};\n" +
"/** @type {number|string} */ T.prototype.x = '';\n" +
"function u() {}\n" +
"/** @param {!T} t\n@return {number} */ function f(t) {\n" +
"if (u()) { t.x = 1; } else { t.x = 2; } return t.x;\n}");
}
public void testDfa9() throws Exception {
testTypes("function f() {\n/** @type {string?} */var x;\nx = null;\n" +
"if (x == null) { return 0; } else { return 1; } }",
"condition always evaluates to true\n" +
"left : null\n" +
"right: null");
}
public void testDfa10() throws Exception {
testTypes("/** @param {null} x */ function g(x) {}" +
"/** @param {string?} x */function f(x) {\n" +
"if (!x) { x = ''; }\n" +
"if (g(x)) { return 0; } else { return 1; } }",
"actual parameter 1 of g does not match formal parameter\n" +
"found : string\n" +
"required: null");
}
public void testDfa11() throws Exception {
testTypes("/** @param {string} opt_x\n@return {string} */\n" +
"function f(opt_x) { if (!opt_x) { " +
"throw new Error('x cannot be empty'); } return opt_x; }");
}
public void testDfa12() throws Exception {
testTypes("/** @param {string} x \n * @constructor \n */" +
"var Bar = function(x) {};" +
"/** @param {string} x */ function g(x) { return true; }" +
"/** @param {string|number} opt_x */ " +
"function f(opt_x) { " +
" if (opt_x) { new Bar(g(opt_x) && 'x'); }" +
"}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : (number|string)\n" +
"required: string");
}
public void testDfa13() throws Exception {
testTypes(
"/**\n" +
" * @param {string} x \n" +
" * @param {number} y \n" +
" * @param {number} z \n" +
" */" +
"function g(x, y, z) {}" +
"function f() { " +
" var x = 'a'; g(x, x = 3, x);" +
"}");
}
public void testTypeInferenceWithCast1() throws Exception {
testTypes(
"/**@return {(number,null,undefined)}*/function u(x) {return null;}" +
"/**@param {number?} x\n@return {number?}*/function f(x) {return x;}" +
"/**@return {number?}*/function g(x) {" +
"var y = /**@type {number?}*/(u(x)); return f(y);}");
}
public void testTypeInferenceWithCast2() throws Exception {
testTypes(
"/**@return {(number,null,undefined)}*/function u(x) {return null;}" +
"/**@param {number?} x\n@return {number?}*/function f(x) {return x;}" +
"/**@return {number?}*/function g(x) {" +
"var y; y = /**@type {number?}*/(u(x)); return f(y);}");
}
public void testTypeInferenceWithCast3() throws Exception {
testTypes(
"/**@return {(number,null,undefined)}*/function u(x) {return 1;}" +
"/**@return {number}*/function g(x) {" +
"return /**@type {number}*/(u(x));}");
}
public void testTypeInferenceWithCast4() throws Exception {
testTypes(
"/**@return {(number,null,undefined)}*/function u(x) {return 1;}" +
"/**@return {number}*/function g(x) {" +
"return /**@type {number}*/(u(x)) && 1;}");
}
public void testTypeInferenceWithCast5() throws Exception {
testTypes(
"/** @param {number} x */ function foo(x) {}" +
"/** @param {{length:*}} y */ function bar(y) {" +
" /** @type {string} */ y.length;" +
" foo(y.length);" +
"}",
"actual parameter 1 of foo does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testTypeInferenceWithClosure1() throws Exception {
testTypes(
"/** @return {boolean} */" +
"function f() {" +
" /** @type {?string} */ var x = null;" +
" function g() { x = 'y'; } g(); " +
" return x == null;" +
"}");
}
public void testTypeInferenceWithClosure2() throws Exception {
testTypes(
"/** @return {boolean} */" +
"function f() {" +
" /** @type {?string} */ var x = null;" +
" function g() { x = 'y'; } g(); " +
" return x === 3;" +
"}",
"condition always evaluates to the same value\n" +
"left : (null|string)\n" +
"right: number");
}
public void testTypeInferenceWithNoEntry1() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"/** @constructor */ function Foo() {}" +
"Foo.prototype.init = function() {" +
" /** @type {?{baz: number}} */ this.bar = {baz: 3};" +
"};" +
"/**\n" +
" * @extends {Foo}\n" +
" * @constructor\n" +
" */" +
"function SubFoo() {}" +
"/** Method */" +
"SubFoo.prototype.method = function() {" +
" for (var i = 0; i < 10; i++) {" +
" f(this.bar);" +
" f(this.bar.baz);" +
" }" +
"};",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (null|{baz: number})\n" +
"required: number");
}
public void testTypeInferenceWithNoEntry2() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @param {number} x */ function f(x) {}" +
"/** @param {!Object} x */ function g(x) {}" +
"/** @constructor */ function Foo() {}" +
"Foo.prototype.init = function() {" +
" /** @type {?{baz: number}} */ this.bar = {baz: 3};" +
"};" +
"/**\n" +
" * @extends {Foo}\n" +
" * @constructor\n" +
" */" +
"function SubFoo() {}" +
"/** Method */" +
"SubFoo.prototype.method = function() {" +
" for (var i = 0; i < 10; i++) {" +
" f(this.bar);" +
" goog.asserts.assert(this.bar);" +
" g(this.bar);" +
" }" +
"};",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (null|{baz: number})\n" +
"required: number");
}
public void testForwardPropertyReference() throws Exception {
testTypes("/** @constructor */ var Foo = function() { this.init(); };" +
"/** @return {string} */" +
"Foo.prototype.getString = function() {" +
" return this.number_;" +
"};" +
"Foo.prototype.init = function() {" +
" /** @type {number} */" +
" this.number_ = 3;" +
"};",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testNoForwardTypeDeclaration() throws Exception {
testTypes(
"/** @param {MyType} x */ function f(x) {}",
"Bad type annotation. Unknown type MyType");
}
public void testNoForwardTypeDeclarationAndNoBraces() throws Exception {
testTypes("/** @return The result. */ function f() {}");
}
public void testForwardTypeDeclaration1() throws Exception {
testClosureTypes(
// malformed addDependency calls shouldn't cause a crash
"goog.addDependency();" +
"goog.addDependency('y', [goog]);" +
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/** @param {MyType} x \n * @return {number} */" +
"function f(x) { return 3; }", null);
}
public void testForwardTypeDeclaration2() throws Exception {
String f = "goog.addDependency('zzz.js', ['MyType'], []);" +
"/** @param {MyType} x */ function f(x) { }";
testClosureTypes(f, null);
testClosureTypes(f + "f(3);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: (MyType|null)");
}
public void testForwardTypeDeclaration3() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/** @param {MyType} x */ function f(x) { return x; }" +
"/** @constructor */ var MyType = function() {};" +
"f(3);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: (MyType|null)");
}
public void testForwardTypeDeclaration4() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/** @param {MyType} x */ function f(x) { return x; }" +
"/** @constructor */ var MyType = function() {};" +
"f(new MyType());",
null);
}
public void testForwardTypeDeclaration5() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/**\n" +
" * @constructor\n" +
" * @extends {MyType}\n" +
" */ var YourType = function() {};" +
"/** @override */ YourType.prototype.method = function() {};",
"Could not resolve type in @extends tag of YourType");
}
public void testForwardTypeDeclaration6() throws Exception {
testClosureTypesMultipleWarnings(
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/**\n" +
" * @constructor\n" +
" * @implements {MyType}\n" +
" */ var YourType = function() {};" +
"/** @override */ YourType.prototype.method = function() {};",
Lists.newArrayList(
"Could not resolve type in @implements tag of YourType",
"property method not defined on any superclass of YourType"));
}
public void testForwardTypeDeclaration7() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/** @param {MyType=} x */" +
"function f(x) { return x == undefined; }", null);
}
public void testForwardTypeDeclaration8() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/** @param {MyType} x */" +
"function f(x) { return x.name == undefined; }", null);
}
public void testForwardTypeDeclaration9() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/** @param {MyType} x */" +
"function f(x) { x.name = 'Bob'; }", null);
}
public void testForwardTypeDeclaration10() throws Exception {
String f = "goog.addDependency('zzz.js', ['MyType'], []);" +
"/** @param {MyType|number} x */ function f(x) { }";
testClosureTypes(f, null);
testClosureTypes(f + "f(3);", null);
testClosureTypes(f + "f('3');",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: (MyType|null|number)");
}
public void testDuplicateTypeDef() throws Exception {
testTypes(
"var goog = {};" +
"/** @constructor */ goog.Bar = function() {};" +
"/** @typedef {number} */ goog.Bar;",
"variable goog.Bar redefined with type None, " +
"original definition at [testcode]:1 " +
"with type function (new:goog.Bar): undefined");
}
public void testTypeDef1() throws Exception {
testTypes(
"var goog = {};" +
"/** @typedef {number} */ goog.Bar;" +
"/** @param {goog.Bar} x */ function f(x) {}" +
"f(3);");
}
public void testTypeDef2() throws Exception {
testTypes(
"var goog = {};" +
"/** @typedef {number} */ goog.Bar;" +
"/** @param {goog.Bar} x */ function f(x) {}" +
"f('3');",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testTypeDef3() throws Exception {
testTypes(
"var goog = {};" +
"/** @typedef {number} */ var Bar;" +
"/** @param {Bar} x */ function f(x) {}" +
"f('3');",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testTypeDef4() throws Exception {
testTypes(
"/** @constructor */ function A() {}" +
"/** @constructor */ function B() {}" +
"/** @typedef {(A|B)} */ var AB;" +
"/** @param {AB} x */ function f(x) {}" +
"f(new A()); f(new B()); f(1);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: (A|B|null)");
}
public void testTypeDef5() throws Exception {
// Notice that the error message is slightly different than
// the one for testTypeDef4, even though they should be the same.
// This is an implementation detail necessary for NamedTypes work out
// ok, and it should change if NamedTypes ever go away.
testTypes(
"/** @param {AB} x */ function f(x) {}" +
"/** @constructor */ function A() {}" +
"/** @constructor */ function B() {}" +
"/** @typedef {(A|B)} */ var AB;" +
"f(new A()); f(new B()); f(1);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: (A|B|null)");
}
public void testCircularTypeDef() throws Exception {
testTypes(
"var goog = {};" +
"/** @typedef {number|Array.<goog.Bar>} */ goog.Bar;" +
"/** @param {goog.Bar} x */ function f(x) {}" +
"f(3); f([3]); f([[3]]);");
}
public void testGetTypedPercent1() throws Exception {
String js = "var id = function(x) { return x; }\n" +
"var id2 = function(x) { return id(x); }";
assertEquals(50.0, getTypedPercent(js), 0.1);
}
public void testGetTypedPercent2() throws Exception {
String js = "var x = {}; x.y = 1;";
assertEquals(100.0, getTypedPercent(js), 0.1);
}
public void testGetTypedPercent3() throws Exception {
String js = "var f = function(x) { x.a = x.b; }";
assertEquals(50.0, getTypedPercent(js), 0.1);
}
public void testGetTypedPercent4() throws Exception {
String js = "var n = {};\n /** @constructor */ n.T = function() {};\n" +
"/** @type n.T */ var x = new n.T();";
assertEquals(100.0, getTypedPercent(js), 0.1);
}
public void testGetTypedPercent5() throws Exception {
String js = "/** @enum {number} */ keys = {A: 1,B: 2,C: 3};";
assertEquals(100.0, getTypedPercent(js), 0.1);
}
public void testGetTypedPercent6() throws Exception {
String js = "a = {TRUE: 1, FALSE: 0};";
assertEquals(100.0, getTypedPercent(js), 0.1);
}
private double getTypedPercent(String js) throws Exception {
Node n = compiler.parseTestCode(js);
Node externs = new Node(Token.BLOCK);
Node externAndJsRoot = new Node(Token.BLOCK, externs, n);
externAndJsRoot.setIsSyntheticBlock(true);
TypeCheck t = makeTypeCheck();
t.processForTesting(null, n);
return t.getTypedPercent();
}
private ObjectType getInstanceType(Node js1Node) {
JSType type = js1Node.getFirstChild().getJSType();
assertNotNull(type);
assertTrue(type instanceof FunctionType);
FunctionType functionType = (FunctionType) type;
assertTrue(functionType.isConstructor());
return functionType.getInstanceType();
}
public void testPrototypePropertyReference() throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope(""
+ "/** @constructor */\n"
+ "function Foo() {}\n"
+ "/** @param {number} a */\n"
+ "Foo.prototype.bar = function(a){};\n"
+ "/** @param {Foo} f */\n"
+ "function baz(f) {\n"
+ " Foo.prototype.bar.call(f, 3);\n"
+ "}");
assertEquals(0, compiler.getErrorCount());
assertEquals(0, compiler.getWarningCount());
assertTrue(p.scope.getVar("Foo").getType() instanceof FunctionType);
FunctionType fooType = (FunctionType) p.scope.getVar("Foo").getType();
assertEquals("function (this:Foo, number): undefined",
fooType.getPrototype().getPropertyType("bar").toString());
}
public void testResolvingNamedTypes() throws Exception {
String js = ""
+ "/** @constructor */\n"
+ "var Foo = function() {}\n"
+ "/** @param {number} a */\n"
+ "Foo.prototype.foo = function(a) {\n"
+ " return this.baz().toString();\n"
+ "};\n"
+ "/** @return {Baz} */\n"
+ "Foo.prototype.baz = function() { return new Baz(); };\n"
+ "/** @constructor\n"
+ " * @extends Foo */\n"
+ "var Bar = function() {};"
+ "/** @constructor */\n"
+ "var Baz = function() {};";
assertEquals(100.0, getTypedPercent(js), 0.1);
}
public void testMissingProperty1() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() { return this.a; };" +
"Foo.prototype.baz = function() { this.a = 3; };");
}
public void testMissingProperty2() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() { return this.a; };" +
"Foo.prototype.baz = function() { this.b = 3; };",
"Property a never defined on Foo");
}
public void testMissingProperty3() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() { return this.a; };" +
"(new Foo).a = 3;");
}
public void testMissingProperty4() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() { return this.a; };" +
"(new Foo).b = 3;",
"Property a never defined on Foo");
}
public void testMissingProperty5() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() { return this.a; };" +
"/** @constructor */ function Bar() { this.a = 3; };",
"Property a never defined on Foo");
}
public void testMissingProperty6() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() { return this.a; };" +
"/** @constructor \n * @extends {Foo} */ " +
"function Bar() { this.a = 3; };");
}
public void testMissingProperty7() throws Exception {
testTypes(
"/** @param {Object} obj */" +
"function foo(obj) { return obj.impossible; }",
"Property impossible never defined on Object");
}
public void testMissingProperty8() throws Exception {
testTypes(
"/** @param {Object} obj */" +
"function foo(obj) { return typeof obj.impossible; }");
}
public void testMissingProperty9() throws Exception {
testTypes(
"/** @param {Object} obj */" +
"function foo(obj) { if (obj.impossible) { return true; } }");
}
public void testMissingProperty10() throws Exception {
testTypes(
"/** @param {Object} obj */" +
"function foo(obj) { while (obj.impossible) { return true; } }");
}
public void testMissingProperty11() throws Exception {
testTypes(
"/** @param {Object} obj */" +
"function foo(obj) { for (;obj.impossible;) { return true; } }");
}
public void testMissingProperty12() throws Exception {
testTypes(
"/** @param {Object} obj */" +
"function foo(obj) { do { } while (obj.impossible); }");
}
public void testMissingProperty13() throws Exception {
testTypes(
"var goog = {}; goog.isDef = function(x) { return false; };" +
"/** @param {Object} obj */" +
"function foo(obj) { return goog.isDef(obj.impossible); }");
}
public void testMissingProperty14() throws Exception {
testTypes(
"var goog = {}; goog.isDef = function(x) { return false; };" +
"/** @param {Object} obj */" +
"function foo(obj) { return goog.isNull(obj.impossible); }",
"Property isNull never defined on goog");
}
public void testMissingProperty15() throws Exception {
testTypes(
"/** @param {Object} x */" +
"function f(x) { if (x.foo) { x.foo(); } }");
}
public void testMissingProperty16() throws Exception {
testTypes(
"/** @param {Object} x */" +
"function f(x) { x.foo(); if (x.foo) {} }",
"Property foo never defined on Object");
}
public void testMissingProperty17() throws Exception {
testTypes(
"/** @param {Object} x */" +
"function f(x) { if (typeof x.foo == 'function') { x.foo(); } }");
}
public void testMissingProperty18() throws Exception {
testTypes(
"/** @param {Object} x */" +
"function f(x) { if (x.foo instanceof Function) { x.foo(); } }");
}
public void testMissingProperty19() throws Exception {
testTypes(
"/** @param {Object} x */" +
"function f(x) { if (x.bar) { if (x.foo) {} } else { x.foo(); } }",
"Property foo never defined on Object");
}
public void testMissingProperty20() throws Exception {
// NOTE(nicksantos): In the else branch, we know that x.foo is a
// CHECKED_UNKNOWN (UNKNOWN restricted to a falsey value). We could
// do some more sophisticated analysis here. Obviously, if x.foo is false,
// then x.foo cannot possibly be called. For example, you could imagine a
// VagueType that was like UnknownType, but had some constraints on it
// so that we knew it could never be a function.
//
// For now, we just punt on this issue.
testTypes(
"/** @param {Object} x */" +
"function f(x) { if (x.foo) { } else { x.foo(); } }");
}
public void testMissingProperty21() throws Exception {
testTypes(
"/** @param {Object} x */" +
"function f(x) { x.foo && x.foo(); }");
}
public void testMissingProperty22() throws Exception {
testTypes(
"/** @param {Object} x \n * @return {boolean} */" +
"function f(x) { return x.foo ? x.foo() : true; }");
}
public void testMissingProperty23() throws Exception {
testTypes(
"function f(x) { x.impossible(); }",
"Property impossible never defined on x");
}
public void testMissingProperty24() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MissingType'], []);" +
"/** @param {MissingType} x */" +
"function f(x) { x.impossible(); }", null);
}
public void testMissingProperty25() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {};" +
"Foo.prototype.bar = function() {};" +
"/** @constructor */ var FooAlias = Foo;" +
"(new FooAlias()).bar();");
}
public void testMissingProperty26() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {};" +
"/** @constructor */ var FooAlias = Foo;" +
"FooAlias.prototype.bar = function() {};" +
"(new Foo()).bar();");
}
public void testMissingProperty27() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MissingType'], []);" +
"/** @param {?MissingType} x */" +
"function f(x) {" +
" for (var parent = x; parent; parent = parent.getParent()) {}" +
"}", null);
}
public void testMissingProperty28() throws Exception {
testTypes(
"function f(obj) {" +
" /** @type {*} */ obj.foo;" +
" return obj.foo;" +
"}");
testTypes(
"function f(obj) {" +
" /** @type {*} */ obj.foo;" +
" return obj.foox;" +
"}",
"Property foox never defined on obj");
}
public void testMissingProperty29() throws Exception {
// This used to emit a warning.
testTypes(
// externs
"/** @constructor */ var Foo;" +
"Foo.prototype.opera;" +
"Foo.prototype.opera.postError;",
"",
null,
false);
}
public void testMissingProperty30() throws Exception {
testTypes(
"/** @return {*} */" +
"function f() {" +
" return {};" +
"}" +
"f().a = 3;" +
"/** @param {Object} y */ function g(y) { return y.a; }");
}
public void testMissingProperty31() throws Exception {
testTypes(
"/** @return {Array|number} */" +
"function f() {" +
" return [];" +
"}" +
"f().a = 3;" +
"/** @param {Array} y */ function g(y) { return y.a; }");
}
public void testMissingProperty32() throws Exception {
testTypes(
"/** @return {Array|number} */" +
"function f() {" +
" return [];" +
"}" +
"f().a = 3;" +
"/** @param {Date} y */ function g(y) { return y.a; }",
"Property a never defined on Date");
}
public void testMissingProperty33() throws Exception {
testTypes(
"/** @param {Object} x */" +
"function f(x) { !x.foo || x.foo(); }");
}
public void testMissingProperty34() throws Exception {
testTypes(
"/** @fileoverview \n * @suppress {missingProperties} */" +
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() { return this.a; };" +
"Foo.prototype.baz = function() { this.b = 3; };");
}
public void testMissingProperty35() throws Exception {
// Bar has specialProp defined, so Bar|Baz may have specialProp defined.
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @constructor */ function Bar() {}" +
"/** @constructor */ function Baz() {}" +
"/** @param {Foo|Bar} x */ function f(x) { x.specialProp = 1; }" +
"/** @param {Bar|Baz} x */ function g(x) { return x.specialProp; }");
}
public void testMissingProperty36() throws Exception {
// Foo has baz defined, and SubFoo has bar defined, so some objects with
// bar may have baz.
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.baz = 0;" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"SubFoo.prototype.bar = 0;" +
"/** @param {{bar: number}} x */ function f(x) { return x.baz; }");
}
public void testMissingProperty37() throws Exception {
// This used to emit a missing property warning because we couldn't
// determine that the inf(Foo, {isVisible:boolean}) == SubFoo.
testTypes(
"/** @param {{isVisible: boolean}} x */ function f(x){" +
" x.isVisible = false;" +
"}" +
"/** @constructor */ function Foo() {}" +
"/**\n" +
" * @constructor \n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/** @type {boolean} */ SubFoo.prototype.isVisible = true;" +
"/**\n" +
" * @param {Foo} x\n" +
" * @return {boolean}\n" +
" */\n" +
"function g(x) { return x.isVisible; }");
}
public void testMissingProperty38() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @constructor */ function Bar() {}" +
"/** @return {Foo|Bar} */ function f() { return new Foo(); }" +
"f().missing;",
"Property missing never defined on (Bar|Foo|null)");
}
public void testMissingProperty39() throws Exception {
testTypes(
"/** @return {string|number} */ function f() { return 3; }" +
"f().length;");
}
public void testMissingProperty40() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MissingType'], []);" +
"/** @param {(Array|MissingType)} x */" +
"function f(x) { x.impossible(); }", null);
}
public void testMissingProperty41() throws Exception {
testTypes(
"/** @param {(Array|Date)} x */" +
"function f(x) { if (x.impossible) x.impossible(); }");
}
public void testReflectObject1() throws Exception {
testClosureTypes(
"var goog = {}; goog.reflect = {}; " +
"goog.reflect.object = function(x, y){};" +
"/** @constructor */ function A() {}" +
"goog.reflect.object(A, {x: 3});",
null);
}
public void testReflectObject2() throws Exception {
testClosureTypes(
"var goog = {}; goog.reflect = {}; " +
"goog.reflect.object = function(x, y){};" +
"/** @param {string} x */ function f(x) {}" +
"/** @constructor */ function A() {}" +
"goog.reflect.object(A, {x: f(1 + 1)});",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testLends1() throws Exception {
testTypes(
"function extend(x, y) {}" +
"/** @constructor */ function Foo() {}" +
"extend(Foo, /** @lends */ ({bar: 1}));",
"Bad type annotation. missing object name in @lends tag");
}
public void testLends2() throws Exception {
testTypes(
"function extend(x, y) {}" +
"/** @constructor */ function Foo() {}" +
"extend(Foo, /** @lends {Foob} */ ({bar: 1}));",
"Variable Foob not declared before @lends annotation.");
}
public void testLends3() throws Exception {
testTypes(
"function extend(x, y) {}" +
"/** @constructor */ function Foo() {}" +
"extend(Foo, {bar: 1});" +
"alert(Foo.bar);",
"Property bar never defined on Foo");
}
public void testLends4() throws Exception {
testTypes(
"function extend(x, y) {}" +
"/** @constructor */ function Foo() {}" +
"extend(Foo, /** @lends {Foo} */ ({bar: 1}));" +
"alert(Foo.bar);");
}
public void testLends5() throws Exception {
testTypes(
"function extend(x, y) {}" +
"/** @constructor */ function Foo() {}" +
"extend(Foo, {bar: 1});" +
"alert((new Foo()).bar);",
"Property bar never defined on Foo");
}
public void testLends6() throws Exception {
testTypes(
"function extend(x, y) {}" +
"/** @constructor */ function Foo() {}" +
"extend(Foo, /** @lends {Foo.prototype} */ ({bar: 1}));" +
"alert((new Foo()).bar);");
}
public void testLends7() throws Exception {
testTypes(
"function extend(x, y) {}" +
"/** @constructor */ function Foo() {}" +
"extend(Foo, /** @lends {Foo.prototype|Foo} */ ({bar: 1}));",
"Bad type annotation. expected closing }");
}
public void testLends8() throws Exception {
testTypes(
"function extend(x, y) {}" +
"/** @type {number} */ var Foo = 3;" +
"extend(Foo, /** @lends {Foo} */ ({bar: 1}));",
"May only lend properties to object types. Foo has type number.");
}
public void testLends9() throws Exception {
testClosureTypesMultipleWarnings(
"function extend(x, y) {}" +
"/** @constructor */ function Foo() {}" +
"extend(Foo, /** @lends {!Foo} */ ({bar: 1}));",
Lists.newArrayList(
"Bad type annotation. expected closing }",
"Bad type annotation. missing object name in @lends tag"));
}
public void testLends10() throws Exception {
testTypes(
"function defineClass(x) { return function() {}; } " +
"/** @constructor */" +
"var Foo = defineClass(" +
" /** @lends {Foo.prototype} */ ({/** @type {number} */ bar: 1}));" +
"/** @return {string} */ function f() { return (new Foo()).bar; }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testLends11() throws Exception {
testTypes(
"function defineClass(x, y) { return function() {}; } " +
"/** @constructor */" +
"var Foo = function() {};" +
"/** @return {*} */ Foo.prototype.bar = function() { return 3; };" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */\n" +
"var SubFoo = defineClass(Foo, " +
" /** @lends {SubFoo.prototype} */ ({\n" +
" /** @return {number} */ bar: function() { return 3; }}));" +
"/** @return {string} */ function f() { return (new SubFoo()).bar(); }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testDeclaredNativeTypeEquality() throws Exception {
Node n = parseAndTypeCheck("/** @constructor */ function Object() {};");
assertEquals(registry.getNativeType(JSTypeNative.OBJECT_FUNCTION_TYPE),
n.getFirstChild().getJSType());
}
public void testUndefinedVar() throws Exception {
Node n = parseAndTypeCheck("var undefined;");
assertEquals(registry.getNativeType(JSTypeNative.VOID_TYPE),
n.getFirstChild().getFirstChild().getJSType());
}
public void testFlowScopeBug1() throws Exception {
Node n = parseAndTypeCheck("/** @param {number} a \n"
+ "* @param {number} b */\n"
+ "function f(a, b) {\n"
+ "/** @type number */"
+ "var i = 0;"
+ "for (; (i + a) < b; ++i) {}}");
// check the type of the add node for i + f
assertEquals(registry.getNativeType(JSTypeNative.NUMBER_TYPE),
n.getFirstChild().getLastChild().getLastChild().getFirstChild()
.getNext().getFirstChild().getJSType());
}
public void testFlowScopeBug2() throws Exception {
Node n = parseAndTypeCheck("/** @constructor */ function Foo() {};\n"
+ "Foo.prototype.hi = false;"
+ "function foo(a, b) {\n"
+ " /** @type Array */"
+ " var arr;"
+ " /** @type number */"
+ " var iter;"
+ " for (iter = 0; iter < arr.length; ++ iter) {"
+ " /** @type Foo */"
+ " var afoo = arr[iter];"
+ " afoo;"
+ " }"
+ "}");
// check the type of afoo when referenced
assertEquals(registry.createNullableType(registry.getType("Foo")),
n.getLastChild().getLastChild().getLastChild().getLastChild()
.getLastChild().getLastChild().getJSType());
}
public void testAddSingletonGetter() {
Node n = parseAndTypeCheck(
"/** @constructor */ function Foo() {};\n" +
"goog.addSingletonGetter(Foo);");
ObjectType o = (ObjectType) n.getFirstChild().getJSType();
assertEquals("function (): Foo",
o.getPropertyType("getInstance").toString());
assertEquals("Foo", o.getPropertyType("instance_").toString());
}
public void testTypeCheckStandaloneAST() throws Exception {
Node n = compiler.parseTestCode("function Foo() { }");
typeCheck(n);
TypedScopeCreator scopeCreator = new TypedScopeCreator(compiler);
Scope topScope = scopeCreator.createScope(n, null);
Node second = compiler.parseTestCode("new Foo");
Node externs = new Node(Token.BLOCK);
Node externAndJsRoot = new Node(Token.BLOCK, externs, second);
externAndJsRoot.setIsSyntheticBlock(true);
new TypeCheck(
compiler,
new SemanticReverseAbstractInterpreter(
compiler.getCodingConvention(), registry),
registry, topScope, scopeCreator, CheckLevel.WARNING, CheckLevel.OFF)
.process(null, second);
assertEquals(1, compiler.getWarningCount());
assertEquals("cannot instantiate non-constructor",
compiler.getWarnings()[0].description);
}
public void testUpdateParameterTypeOnClosure() throws Exception {
testTypes(
"/**\n" +
"* @constructor\n" +
"* @param {*=} opt_value\n" +
"* @return {?}\n" +
"*/\n" +
"function Object(opt_value) {}\n" +
"/**\n" +
"* @constructor\n" +
"* @param {...*} var_args\n" +
"*/\n" +
"function Function(var_args) {}\n" +
"/**\n" +
"* @type {Function}\n" +
"*/\n" +
// The line below sets JSDocInfo on Object so that the type of the
// argument to function f has JSDoc through its prototype chain.
"Object.prototype.constructor = function() {};\n",
"/**\n" +
"* @param {function(): boolean} fn\n" +
"*/\n" +
"function f(fn) {}\n" +
"f(function(g) { });\n",
null,
false);
}
public void testBadTemplateType1() throws Exception {
testTypes(
"/**\n" +
"* @param {T} x\n" +
"* @param {T} y\n" +
"* @param {function(this:T, ...)} z\n" +
"* @template T\n" +
"*/\n" +
"function f(x, y, z) {}\n" +
"f(this, this, function() { this });",
FunctionTypeBuilder.TEMPLATE_TYPE_DUPLICATED.format());
}
public void testBadTemplateType2() throws Exception {
testTypes(
"/**\n" +
"* @param {T} x\n" +
"* @param {function(this:T, ...)} y\n" +
"* @template T\n" +
"*/\n" +
"function f(x, y) {}\n" +
"f(0, function() {});",
TypeInference.TEMPLATE_TYPE_NOT_OBJECT_TYPE.format("number"));
}
public void testBadTemplateType3() throws Exception {
testTypes(
"/**\n" +
" * @param {T} x\n" +
" * @template T\n" +
"*/\n" +
"function f(x) {}\n" +
"f(this);",
TypeInference.TEMPLATE_TYPE_OF_THIS_EXPECTED.format());
}
public void testBadTemplateType4() throws Exception {
testTypes(
"/**\n" +
"* @template T\n" +
"*/\n" +
"function f() {}\n" +
"f();",
FunctionTypeBuilder.TEMPLATE_TYPE_EXPECTED.format());
}
public void testBadTemplateType5() throws Exception {
testTypes(
"/**\n" +
"* @template T\n" +
"* @return {T}\n" +
"*/\n" +
"function f() {}\n" +
"f();",
FunctionTypeBuilder.TEMPLATE_TYPE_EXPECTED.format());
}
public void testFunctionLiteralUndefinedThisArgument() throws Exception {
testTypes(""
+ "/**\n"
+ " * @param {function(this:T, ...)?} fn\n"
+ " * @param {?T} opt_obj\n"
+ " * @template T\n"
+ " */\n"
+ "function baz(fn, opt_obj) {}\n"
+ "baz(function() { this; });",
"Function literal argument refers to undefined this argument");
}
public void testFunctionLiteralDefinedThisArgument() throws Exception {
testTypes(""
+ "/**\n"
+ " * @param {function(this:T, ...)?} fn\n"
+ " * @param {?T} opt_obj\n"
+ " * @template T\n"
+ " */\n"
+ "function baz(fn, opt_obj) {}\n"
+ "baz(function() { this; }, {});");
}
public void testFunctionLiteralUnreadNullThisArgument() throws Exception {
testTypes(""
+ "/**\n"
+ " * @param {function(this:T, ...)?} fn\n"
+ " * @param {?T} opt_obj\n"
+ " * @template T\n"
+ " */\n"
+ "function baz(fn, opt_obj) {}\n"
+ "baz(function() {}, null);");
}
public void testUnionTemplateThisType() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** @return {F|Array} */ function g() { return []; }" +
"/** @param {F} x */ function h(x) { }" +
"/**\n" +
"* @param {T} x\n" +
"* @param {function(this:T, ...)} y\n" +
"* @template T\n" +
"*/\n" +
"function f(x, y) {}\n" +
"f(g(), function() { h(this); });",
"actual parameter 1 of h does not match formal parameter\n" +
"found : Object\n" +
"required: (F|null)");
}
public void testActiveXObject() throws Exception {
testTypes(
"/** @type {Object} */ var x = new ActiveXObject();" +
"/** @type { {impossibleProperty} } */ var y = new ActiveXObject();");
}
public void testRecordType1() throws Exception {
testTypes(
"/** @param {{prop: number}} x */" +
"function f(x) {}" +
"f({});",
"actual parameter 1 of f does not match formal parameter\n" +
"found : {prop: (number|undefined)}\n" +
"required: {prop: number}");
}
public void testRecordType2() throws Exception {
testTypes(
"/** @param {{prop: (number|undefined)}} x */" +
"function f(x) {}" +
"f({});");
}
public void testRecordType3() throws Exception {
testTypes(
"/** @param {{prop: number}} x */" +
"function f(x) {}" +
"f({prop: 'x'});",
"actual parameter 1 of f does not match formal parameter\n" +
"found : {prop: (number|string)}\n" +
"required: {prop: number}");
}
public void testRecordType4() throws Exception {
// Notice that we do not do flow-based inference on the object type:
// We don't try to prove that x.prop may not be string until x
// gets passed to g.
testClosureTypesMultipleWarnings(
"/** @param {{prop: (number|undefined)}} x */" +
"function f(x) {}" +
"/** @param {{prop: (string|undefined)}} x */" +
"function g(x) {}" +
"var x = {}; f(x); g(x);",
Lists.newArrayList(
"actual parameter 1 of f does not match formal parameter\n" +
"found : {prop: (number|string|undefined)}\n" +
"required: {prop: (number|undefined)}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : {prop: (number|string|undefined)}\n" +
"required: {prop: (string|undefined)}"));
}
public void testRecordType5() throws Exception {
testTypes(
"/** @param {{prop: (number|undefined)}} x */" +
"function f(x) {}" +
"/** @param {{otherProp: (string|undefined)}} x */" +
"function g(x) {}" +
"var x = {}; f(x); g(x);");
}
public void testRecordType6() throws Exception {
testTypes(
"/** @return {{prop: (number|undefined)}} x */" +
"function f() { return {}; }");
}
public void testRecordType7() throws Exception {
testTypes(
"/** @return {{prop: (number|undefined)}} x */" +
"function f() { var x = {}; g(x); return x; }" +
"/** @param {number} x */" +
"function g(x) {}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : {prop: (number|undefined)}\n" +
"required: number");
}
public void testRecordType8() throws Exception {
testTypes(
"/** @return {{prop: (number|string)}} x */" +
"function f() { var x = {prop: 3}; g(x.prop); return x; }" +
"/** @param {string} x */" +
"function g(x) {}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testDuplicateRecordFields1() throws Exception {
testTypes("/**"
+ "* @param {{x:string, x:number}} a"
+ "*/"
+ "function f(a) {};",
"Parse error. Duplicate record field x");
}
public void testDuplicateRecordFields2() throws Exception {
testTypes("/**"
+ "* @param {{name:string,number:x,number:y}} a"
+ " */"
+ "function f(a) {};",
new String[] {"Bad type annotation. Unknown type x",
"Parse error. Duplicate record field number",
"Bad type annotation. Unknown type y"});
}
public void testMultipleExtendsInterface1() throws Exception {
testTypes("/** @interface */ function base1() {}\n"
+ "/** @interface */ function base2() {}\n"
+ "/** @interface\n"
+ "* @extends {base1}\n"
+ "* @extends {base2}\n"
+ "*/\n"
+ "function derived() {}");
}
public void testMultipleExtendsInterface2() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @desc description */Int0.prototype.foo = function() {};" +
"/** @interface \n @extends {Int0} \n @extends {Int1} */" +
"function Int2() {};" +
"/** @constructor\n @implements {Int2} */function Foo() {};",
"property foo on interface Int0 is not implemented by type Foo");
}
public void testMultipleExtendsInterface3() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @desc description */Int1.prototype.foo = function() {};" +
"/** @interface \n @extends {Int0} \n @extends {Int1} */" +
"function Int2() {};" +
"/** @constructor\n @implements {Int2} */function Foo() {};",
"property foo on interface Int1 is not implemented by type Foo");
}
public void testMultipleExtendsInterface4() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @interface \n @extends {Int0} \n @extends {Int1} \n" +
" @extends {number} */" +
"function Int2() {};" +
"/** @constructor\n @implements {Int2} */function Foo() {};",
"Int2 @extends non-object type number");
}
public void testMultipleExtendsInterface5() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @constructor */function Int1() {};" +
"/** @desc description @ return {string} x */" +
"/** @interface \n @extends {Int0} \n @extends {Int1} */" +
"function Int2() {};",
"Int2 cannot extend this type; a constructor can only extend " +
"objects and an interface can only extend interfaces");
}
public void testMultipleExtendsInterface6() throws Exception {
testTypes(
"/** @interface */function Super1() {};" +
"/** @interface */function Super2() {};" +
"/** @param {number} bar */Super2.prototype.foo = function(bar) {};" +
"/** @interface\n @extends {Super1}\n " +
"@extends {Super2} */function Sub() {};" +
"/** @override\n @param {string} bar */Sub.prototype.foo =\n" +
"function(bar) {};",
"mismatch of the foo property type and the type of the property it " +
"overrides from superclass Super2\n" +
"original: function (this:Super2, number): undefined\n" +
"override: function (this:Sub, string): undefined");
}
public void testMultipleExtendsInterfaceAssignment() throws Exception {
testTypes("/** @interface */var I1 = function() {};\n" +
"/** @interface */ var I2 = function() {}\n" +
"/** @interface\n@extends {I1}\n@extends {I2}*/" +
"var I3 = function() {};\n" +
"/** @constructor\n@implements {I3}*/var T = function() {};\n" +
"var t = new T();\n" +
"/** @type {I1} */var i1 = t;\n" +
"/** @type {I2} */var i2 = t;\n" +
"/** @type {I3} */var i3 = t;\n" +
"i1 = i3;\n" +
"i2 = i3;\n");
}
public void testMultipleExtendsInterfaceParamPass() throws Exception {
testTypes("/** @interface */var I1 = function() {};\n" +
"/** @interface */ var I2 = function() {}\n" +
"/** @interface\n@extends {I1}\n@extends {I2}*/" +
"var I3 = function() {};\n" +
"/** @constructor\n@implements {I3}*/var T = function() {};\n" +
"var t = new T();\n" +
"/** @param x I1 \n@param y I2\n@param z I3*/function foo(x,y,z){};\n" +
"foo(t,t,t)\n");
}
public void testBadMultipleExtendsClass() throws Exception {
testTypes("/** @constructor */ function base1() {}\n"
+ "/** @constructor */ function base2() {}\n"
+ "/** @constructor\n"
+ "* @extends {base1}\n"
+ "* @extends {base2}\n"
+ "*/\n"
+ "function derived() {}",
"Bad type annotation. type annotation incompatible "
+ "with other annotations");
}
public void testInterfaceExtendsResolution() throws Exception {
testTypes("/** @interface \n @extends {A} */ function B() {};\n" +
"/** @constructor \n @implements {B} */ function C() {};\n" +
"/** @interface */ function A() {};");
}
public void testPropertyCanBeDefinedInObject() throws Exception {
testTypes("/** @interface */ function I() {};" +
"I.prototype.bar = function() {};" +
"/** @type {Object} */ var foo;" +
"foo.bar();");
}
private void checkObjectType(ObjectType objectType, String propertyName,
JSType expectedType) {
assertTrue("Expected " + objectType.getReferenceName() +
" to have property " +
propertyName, objectType.hasProperty(propertyName));
assertEquals("Expected " + objectType.getReferenceName() +
"'s property " +
propertyName + " to have type " + expectedType,
expectedType, objectType.getPropertyType(propertyName));
}
public void testExtendedInterfacePropertiesCompatibility1() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @type {number} */" +
"Int0.prototype.foo;" +
"/** @type {string} */" +
"Int1.prototype.foo;" +
"/** @interface \n @extends {Int0} \n @extends {Int1} */" +
"function Int2() {};",
"Interface Int2 has a property foo with incompatible types in its " +
"super interfaces Int0 and Int1");
}
public void testExtendedInterfacePropertiesCompatibility2() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @interface */function Int2() {};" +
"/** @type {number} */" +
"Int0.prototype.foo;" +
"/** @type {string} */" +
"Int1.prototype.foo;" +
"/** @type {Object} */" +
"Int2.prototype.foo;" +
"/** @interface \n @extends {Int0} \n @extends {Int1} \n" +
"@extends {Int2}*/" +
"function Int3() {};",
new String[] {
"Interface Int3 has a property foo with incompatible types in " +
"its super interfaces Int0 and Int1",
"Interface Int3 has a property foo with incompatible types in " +
"its super interfaces Int1 and Int2"
});
}
public void testExtendedInterfacePropertiesCompatibility3() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @type {number} */" +
"Int0.prototype.foo;" +
"/** @type {string} */" +
"Int1.prototype.foo;" +
"/** @interface \n @extends {Int1} */ function Int2() {};" +
"/** @interface \n @extends {Int0} \n @extends {Int2} */" +
"function Int3() {};",
"Interface Int3 has a property foo with incompatible types in its " +
"super interfaces Int0 and Int1");
}
public void testExtendedInterfacePropertiesCompatibility4() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface \n @extends {Int0} */ function Int1() {};" +
"/** @type {number} */" +
"Int0.prototype.foo;" +
"/** @interface */function Int2() {};" +
"/** @interface \n @extends {Int2} */ function Int3() {};" +
"/** @type {string} */" +
"Int2.prototype.foo;" +
"/** @interface \n @extends {Int1} \n @extends {Int3} */" +
"function Int4() {};",
"Interface Int4 has a property foo with incompatible types in its " +
"super interfaces Int0 and Int2");
}
public void testExtendedInterfacePropertiesCompatibility5() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @type {number} */" +
"Int0.prototype.foo;" +
"/** @type {string} */" +
"Int1.prototype.foo;" +
"/** @interface \n @extends {Int1} */ function Int2() {};" +
"/** @interface \n @extends {Int0} \n @extends {Int2} */" +
"function Int3() {};" +
"/** @interface */function Int4() {};" +
"/** @type {number} */" +
"Int4.prototype.foo;" +
"/** @interface \n @extends {Int3} \n @extends {Int4} */" +
"function Int5() {};",
new String[] {
"Interface Int3 has a property foo with incompatible types in its" +
" super interfaces Int0 and Int1",
"Interface Int5 has a property foo with incompatible types in its" +
" super interfaces Int1 and Int4"});
}
public void testExtendedInterfacePropertiesCompatibility6() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @type {number} */" +
"Int0.prototype.foo;" +
"/** @type {string} */" +
"Int1.prototype.foo;" +
"/** @interface \n @extends {Int1} */ function Int2() {};" +
"/** @interface \n @extends {Int0} \n @extends {Int2} */" +
"function Int3() {};" +
"/** @interface */function Int4() {};" +
"/** @type {string} */" +
"Int4.prototype.foo;" +
"/** @interface \n @extends {Int3} \n @extends {Int4} */" +
"function Int5() {};",
"Interface Int3 has a property foo with incompatible types in its" +
" super interfaces Int0 and Int1");
}
public void testExtendedInterfacePropertiesCompatibility7() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @type {number} */" +
"Int0.prototype.foo;" +
"/** @type {string} */" +
"Int1.prototype.foo;" +
"/** @interface \n @extends {Int1} */ function Int2() {};" +
"/** @interface \n @extends {Int0} \n @extends {Int2} */" +
"function Int3() {};" +
"/** @interface */function Int4() {};" +
"/** @type {Object} */" +
"Int4.prototype.foo;" +
"/** @interface \n @extends {Int3} \n @extends {Int4} */" +
"function Int5() {};",
new String[] {
"Interface Int3 has a property foo with incompatible types in its" +
" super interfaces Int0 and Int1",
"Interface Int5 has a property foo with incompatible types in its" +
" super interfaces Int1 and Int4"});
}
public void testExtendedInterfacePropertiesCompatibility8() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @type {number} */" +
"Int0.prototype.foo;" +
"/** @type {string} */" +
"Int1.prototype.bar;" +
"/** @interface \n @extends {Int1} */ function Int2() {};" +
"/** @interface \n @extends {Int0} \n @extends {Int2} */" +
"function Int3() {};" +
"/** @interface */function Int4() {};" +
"/** @type {Object} */" +
"Int4.prototype.foo;" +
"/** @type {Null} */" +
"Int4.prototype.bar;" +
"/** @interface \n @extends {Int3} \n @extends {Int4} */" +
"function Int5() {};",
new String[] {
"Interface Int5 has a property bar with incompatible types in its" +
" super interfaces Int1 and Int4",
"Interface Int5 has a property foo with incompatible types in its" +
" super interfaces Int0 and Int4"});
}
private void testTypes(String js) throws Exception {
testTypes(js, (String) null);
}
private void testTypes(String js, String description) throws Exception {
testTypes(js, description, false);
}
private void testTypes(String js, DiagnosticType type) throws Exception {
testTypes(js, type.format(), false);
}
private void testClosureTypes(String js, String description)
throws Exception {
testClosureTypesMultipleWarnings(js,
description == null ? null : Lists.newArrayList(description));
}
private void testClosureTypesMultipleWarnings(
String js, List<String> descriptions) throws Exception {
Node n = compiler.parseTestCode(js);
Node externs = new Node(Token.BLOCK);
Node externAndJsRoot = new Node(Token.BLOCK, externs, n);
externAndJsRoot.setIsSyntheticBlock(true);
assertEquals("parsing error: " +
Joiner.on(", ").join(compiler.getErrors()),
0, compiler.getErrorCount());
// For processing goog.addDependency for forward typedefs.
new ProcessClosurePrimitives(compiler, null, CheckLevel.ERROR, true)
.process(null, n);
CodingConvention convention = compiler.getCodingConvention();
new TypeCheck(compiler,
new ClosureReverseAbstractInterpreter(
convention, registry).append(
new SemanticReverseAbstractInterpreter(
convention, registry))
.getFirst(),
registry)
.processForTesting(null, n);
assertEquals(
"unexpected error(s) : " +
Joiner.on(", ").join(compiler.getErrors()),
0, compiler.getErrorCount());
if (descriptions == null) {
assertEquals(
"unexpected warning(s) : " +
Joiner.on(", ").join(compiler.getWarnings()),
0, compiler.getWarningCount());
} else {
assertEquals(
"unexpected warning(s) : " +
Joiner.on(", ").join(compiler.getWarnings()),
descriptions.size(), compiler.getWarningCount());
for (int i = 0; i < descriptions.size(); i++) {
assertEquals(descriptions.get(i),
compiler.getWarnings()[i].description);
}
}
}
void testTypes(String js, String description, boolean isError)
throws Exception {
testTypes(DEFAULT_EXTERNS, js, description, isError);
}
void testTypes(String externs, String js, String description, boolean isError)
throws Exception {
Node n = parseAndTypeCheck(externs, js);
JSError[] errors = compiler.getErrors();
if (description != null && isError) {
assertTrue("expected an error", errors.length > 0);
assertEquals(description, errors[0].description);
errors = Arrays.asList(errors).subList(1, errors.length).toArray(
new JSError[errors.length - 1]);
}
if (errors.length > 0) {
fail("unexpected error(s):\n" + Joiner.on("\n").join(errors));
}
JSError[] warnings = compiler.getWarnings();
if (description != null && !isError) {
assertTrue("expected a warning", warnings.length > 0);
assertEquals(description, warnings[0].description);
warnings = Arrays.asList(warnings).subList(1, warnings.length).toArray(
new JSError[warnings.length - 1]);
}
if (warnings.length > 0) {
fail("unexpected warnings(s):\n" + Joiner.on("\n").join(warnings));
}
}
/**
* Parses and type checks the JavaScript code.
*/
private Node parseAndTypeCheck(String js) {
return parseAndTypeCheck(DEFAULT_EXTERNS, js);
}
private Node parseAndTypeCheck(String externs, String js) {
return parseAndTypeCheckWithScope(externs, js).root;
}
/**
* Parses and type checks the JavaScript code and returns the Scope used
* whilst type checking.
*/
private TypeCheckResult parseAndTypeCheckWithScope(String js) {
return parseAndTypeCheckWithScope(DEFAULT_EXTERNS, js);
}
private TypeCheckResult parseAndTypeCheckWithScope(
String externs, String js) {
compiler.init(
Lists.newArrayList(SourceFile.fromCode("[externs]", externs)),
Lists.newArrayList(SourceFile.fromCode("[testcode]", js)),
compiler.getOptions());
Node n = compiler.getInput(new InputId("[testcode]")).getAstRoot(compiler);
Node externsNode = compiler.getInput(new InputId("[externs]"))
.getAstRoot(compiler);
Node externAndJsRoot = new Node(Token.BLOCK, externsNode, n);
externAndJsRoot.setIsSyntheticBlock(true);
assertEquals("parsing error: " +
Joiner.on(", ").join(compiler.getErrors()),
0, compiler.getErrorCount());
Scope s = makeTypeCheck().processForTesting(externsNode, n);
return new TypeCheckResult(n, s);
}
private Node typeCheck(Node n) {
Node externsNode = new Node(Token.BLOCK);
Node externAndJsRoot = new Node(Token.BLOCK, externsNode, n);
externAndJsRoot.setIsSyntheticBlock(true);
makeTypeCheck().processForTesting(null, n);
return n;
}
private TypeCheck makeTypeCheck() {
return new TypeCheck(
compiler,
new SemanticReverseAbstractInterpreter(
compiler.getCodingConvention(), registry),
registry,
reportMissingOverrides,
CheckLevel.OFF);
}
void testTypes(String js, String[] warnings) throws Exception {
Node n = compiler.parseTestCode(js);
assertEquals(0, compiler.getErrorCount());
Node externsNode = new Node(Token.BLOCK);
Node externAndJsRoot = new Node(Token.BLOCK, externsNode, n);
makeTypeCheck().processForTesting(null, n);
assertEquals(0, compiler.getErrorCount());
if (warnings != null) {
assertEquals(warnings.length, compiler.getWarningCount());
JSError[] messages = compiler.getWarnings();
for (int i = 0; i < warnings.length && i < compiler.getWarningCount();
i++) {
assertEquals(warnings[i], messages[i].description);
}
} else {
assertEquals(0, compiler.getWarningCount());
}
}
String suppressMissingProperty(String ... props) {
String result = "function dummy(x) { ";
for (String prop : props) {
result += "x." + prop + " = 3;";
}
return result + "}";
}
private static class TypeCheckResult {
private final Node root;
private final Scope scope;
private TypeCheckResult(Node root, Scope scope) {
this.root = root;
this.scope = scope;
}
}
} | // You are a professional Java test case writer, please create a test case named `testIssue700` for the issue `Closure-700`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-700
//
// ## Issue-Title:
// weird object literal invalid property error on unrelated object prototype
//
// ## Issue-Description:
// Apologies in advance for the convoluted repro case and the vague summary.
//
// Compile the following code (attached as repro.js) with:
// java -jar build/compiler.jar --compilation\_level=ADVANCED\_OPTIMIZATIONS --jscomp\_error=accessControls --jscomp\_error=checkTypes --jscomp\_error=checkVars --js repro.js \*
//
// /\*\*
// \* @param {{text: string}} opt\_data
// \* @return {string}
// \*/
// function temp1(opt\_data) {
// return opt\_data.text;
// }
//
// /\*\*
// \* @param {{activity: (boolean|number|string|null|Object)}} opt\_data
// \* @return {string}
// \*/
// function temp2(opt\_data) {
// /\*\* @notypecheck \*/
// function \_\_inner() {
// return temp1(opt\_data.activity);
// }
// return \_\_inner();
// }
//
// /\*\*
// \* @param {{n: number, text: string, b: boolean}} opt\_data
// \* @return {string}
// \*/
// function temp3(opt\_data) {
// return 'n: ' + opt\_data.n + ', t: ' + opt\_data.text + '.';
// }
//
// function callee() {
// var output = temp3({
// n: 0,
// text: 'a string',
// b: true
// })
// alert(output);
// }
//
// callee();
//
//
// yields:
// repro.js:30: ERROR - actual parameter 1 of temp3 does not match formal parameter
// found : {b: boolean, n: number, text: (string|undefined)}
// required: {b: boolean, n: number, text: string}
// var output = temp3({
//
// It seems like temp3 is actually being called with the right type {b: boolean, n: number, text: string} though it seems to think that text is a (string|undefined)
// This seems to happen because of the seemingly unrelated code in functions temp1 and temp2. If I change the name of the text property (as in repro3.js) it works.
// Additionally, if I fix the type of the activity property in the record type of temp2 it works (as in repro2.js)
//
// This comes up in our codebase in some situations where we don't have type info for all the objects being passed into a function. It's always a tricky one to find because it reports an error at a location that looks correct.
//
//
// \* it also fails with SIMPLE\_OPTIMIZATIONS
//
//
public void testIssue700() throws Exception {
| 5,815 | 33 | 5,775 | test/com/google/javascript/jscomp/TypeCheckTest.java | test | ```markdown
## Issue-ID: Closure-700
## Issue-Title:
weird object literal invalid property error on unrelated object prototype
## Issue-Description:
Apologies in advance for the convoluted repro case and the vague summary.
Compile the following code (attached as repro.js) with:
java -jar build/compiler.jar --compilation\_level=ADVANCED\_OPTIMIZATIONS --jscomp\_error=accessControls --jscomp\_error=checkTypes --jscomp\_error=checkVars --js repro.js \*
/\*\*
\* @param {{text: string}} opt\_data
\* @return {string}
\*/
function temp1(opt\_data) {
return opt\_data.text;
}
/\*\*
\* @param {{activity: (boolean|number|string|null|Object)}} opt\_data
\* @return {string}
\*/
function temp2(opt\_data) {
/\*\* @notypecheck \*/
function \_\_inner() {
return temp1(opt\_data.activity);
}
return \_\_inner();
}
/\*\*
\* @param {{n: number, text: string, b: boolean}} opt\_data
\* @return {string}
\*/
function temp3(opt\_data) {
return 'n: ' + opt\_data.n + ', t: ' + opt\_data.text + '.';
}
function callee() {
var output = temp3({
n: 0,
text: 'a string',
b: true
})
alert(output);
}
callee();
yields:
repro.js:30: ERROR - actual parameter 1 of temp3 does not match formal parameter
found : {b: boolean, n: number, text: (string|undefined)}
required: {b: boolean, n: number, text: string}
var output = temp3({
It seems like temp3 is actually being called with the right type {b: boolean, n: number, text: string} though it seems to think that text is a (string|undefined)
This seems to happen because of the seemingly unrelated code in functions temp1 and temp2. If I change the name of the text property (as in repro3.js) it works.
Additionally, if I fix the type of the activity property in the record type of temp2 it works (as in repro2.js)
This comes up in our codebase in some situations where we don't have type info for all the objects being passed into a function. It's always a tricky one to find because it reports an error at a location that looks correct.
\* it also fails with SIMPLE\_OPTIMIZATIONS
```
You are a professional Java test case writer, please create a test case named `testIssue700` for the issue `Closure-700`, utilizing the provided issue report information and the following function signature.
```java
public void testIssue700() throws Exception {
```
| 5,775 | [
"com.google.javascript.rhino.jstype.PrototypeObjectType"
] | 7938ce18334029daf23cb96a1507f8f02ef479515b108bd14e62ffe1e0286089 | public void testIssue700() throws Exception | // You are a professional Java test case writer, please create a test case named `testIssue700` for the issue `Closure-700`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-700
//
// ## Issue-Title:
// weird object literal invalid property error on unrelated object prototype
//
// ## Issue-Description:
// Apologies in advance for the convoluted repro case and the vague summary.
//
// Compile the following code (attached as repro.js) with:
// java -jar build/compiler.jar --compilation\_level=ADVANCED\_OPTIMIZATIONS --jscomp\_error=accessControls --jscomp\_error=checkTypes --jscomp\_error=checkVars --js repro.js \*
//
// /\*\*
// \* @param {{text: string}} opt\_data
// \* @return {string}
// \*/
// function temp1(opt\_data) {
// return opt\_data.text;
// }
//
// /\*\*
// \* @param {{activity: (boolean|number|string|null|Object)}} opt\_data
// \* @return {string}
// \*/
// function temp2(opt\_data) {
// /\*\* @notypecheck \*/
// function \_\_inner() {
// return temp1(opt\_data.activity);
// }
// return \_\_inner();
// }
//
// /\*\*
// \* @param {{n: number, text: string, b: boolean}} opt\_data
// \* @return {string}
// \*/
// function temp3(opt\_data) {
// return 'n: ' + opt\_data.n + ', t: ' + opt\_data.text + '.';
// }
//
// function callee() {
// var output = temp3({
// n: 0,
// text: 'a string',
// b: true
// })
// alert(output);
// }
//
// callee();
//
//
// yields:
// repro.js:30: ERROR - actual parameter 1 of temp3 does not match formal parameter
// found : {b: boolean, n: number, text: (string|undefined)}
// required: {b: boolean, n: number, text: string}
// var output = temp3({
//
// It seems like temp3 is actually being called with the right type {b: boolean, n: number, text: string} though it seems to think that text is a (string|undefined)
// This seems to happen because of the seemingly unrelated code in functions temp1 and temp2. If I change the name of the text property (as in repro3.js) it works.
// Additionally, if I fix the type of the activity property in the record type of temp2 it works (as in repro2.js)
//
// This comes up in our codebase in some situations where we don't have type info for all the objects being passed into a function. It's always a tricky one to find because it reports an error at a location that looks correct.
//
//
// \* it also fails with SIMPLE\_OPTIMIZATIONS
//
//
| Closure | /*
* Copyright 2006 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import com.google.common.base.Joiner;
import com.google.common.collect.Lists;
import com.google.javascript.jscomp.Scope.Var;
import com.google.javascript.rhino.InputId;
import com.google.javascript.rhino.Node;
import com.google.javascript.rhino.Token;
import com.google.javascript.rhino.jstype.FunctionType;
import com.google.javascript.rhino.jstype.JSType;
import com.google.javascript.rhino.jstype.JSTypeNative;
import com.google.javascript.rhino.jstype.ObjectType;
import java.util.Arrays;
import java.util.List;
/**
* Tests {@link TypeCheck}.
*
*/
public class TypeCheckTest extends CompilerTypeTestCase {
private CheckLevel reportMissingOverrides = CheckLevel.WARNING;
@Override
public void setUp() throws Exception {
super.setUp();
reportMissingOverrides = CheckLevel.WARNING;
}
public void testInitialTypingScope() {
Scope s = new TypedScopeCreator(compiler,
CodingConventions.getDefault()).createInitialScope(
new Node(Token.BLOCK));
assertEquals(ARRAY_FUNCTION_TYPE, s.getVar("Array").getType());
assertEquals(BOOLEAN_OBJECT_FUNCTION_TYPE,
s.getVar("Boolean").getType());
assertEquals(DATE_FUNCTION_TYPE, s.getVar("Date").getType());
assertEquals(ERROR_FUNCTION_TYPE, s.getVar("Error").getType());
assertEquals(EVAL_ERROR_FUNCTION_TYPE,
s.getVar("EvalError").getType());
assertEquals(NUMBER_OBJECT_FUNCTION_TYPE,
s.getVar("Number").getType());
assertEquals(OBJECT_FUNCTION_TYPE, s.getVar("Object").getType());
assertEquals(RANGE_ERROR_FUNCTION_TYPE,
s.getVar("RangeError").getType());
assertEquals(REFERENCE_ERROR_FUNCTION_TYPE,
s.getVar("ReferenceError").getType());
assertEquals(REGEXP_FUNCTION_TYPE, s.getVar("RegExp").getType());
assertEquals(STRING_OBJECT_FUNCTION_TYPE,
s.getVar("String").getType());
assertEquals(SYNTAX_ERROR_FUNCTION_TYPE,
s.getVar("SyntaxError").getType());
assertEquals(TYPE_ERROR_FUNCTION_TYPE,
s.getVar("TypeError").getType());
assertEquals(URI_ERROR_FUNCTION_TYPE,
s.getVar("URIError").getType());
}
public void testTypeCheck1() throws Exception {
testTypes("/**@return {void}*/function foo(){ if (foo()) return; }");
}
public void testTypeCheck2() throws Exception {
testTypes("/**@return {void}*/function foo(){ var x=foo(); x--; }",
"increment/decrement\n" +
"found : undefined\n" +
"required: number");
}
public void testTypeCheck4() throws Exception {
testTypes("/**@return {void}*/function foo(){ !foo(); }");
}
public void testTypeCheck5() throws Exception {
testTypes("/**@return {void}*/function foo(){ var a = +foo(); }",
"sign operator\n" +
"found : undefined\n" +
"required: number");
}
public void testTypeCheck6() throws Exception {
testTypes(
"/**@return {void}*/function foo(){" +
"/** @type {undefined|number} */var a;if (a == foo())return;}");
}
public void testTypeCheck8() throws Exception {
testTypes("/**@return {void}*/function foo(){do {} while (foo());}");
}
public void testTypeCheck9() throws Exception {
testTypes("/**@return {void}*/function foo(){while (foo());}");
}
public void testTypeCheck10() throws Exception {
testTypes("/**@return {void}*/function foo(){for (;foo(););}");
}
public void testTypeCheck11() throws Exception {
testTypes("/**@type !Number */var a;" +
"/**@type !String */var b;" +
"a = b;",
"assignment\n" +
"found : String\n" +
"required: Number");
}
public void testTypeCheck12() throws Exception {
testTypes("/**@return {!Object}*/function foo(){var a = 3^foo();}",
"bad right operand to bitwise operator\n" +
"found : Object\n" +
"required: (boolean|null|number|string|undefined)");
}
public void testTypeCheck13() throws Exception {
testTypes("/**@type {!Number|!String}*/var i; i=/xx/;",
"assignment\n" +
"found : RegExp\n" +
"required: (Number|String)");
}
public void testTypeCheck14() throws Exception {
testTypes("/**@param opt_a*/function foo(opt_a){}");
}
public void testTypeCheck15() throws Exception {
testTypes("/**@type {Number|null} */var x;x=null;x=10;",
"assignment\n" +
"found : number\n" +
"required: (Number|null)");
}
public void testTypeCheck16() throws Exception {
testTypes("/**@type {Number|null} */var x='';",
"initializing variable\n" +
"found : string\n" +
"required: (Number|null)");
}
public void testTypeCheck17() throws Exception {
testTypes("/**@return {Number}\n@param {Number} opt_foo */\n" +
"function a(opt_foo){\nreturn /**@type {Number}*/(opt_foo);\n}");
}
public void testTypeCheck18() throws Exception {
testTypes("/**@return {RegExp}\n*/\n function a(){return new RegExp();}");
}
public void testTypeCheck19() throws Exception {
testTypes("/**@return {Array}\n*/\n function a(){return new Array();}");
}
public void testTypeCheck20() throws Exception {
testTypes("/**@return {Date}\n*/\n function a(){return new Date();}");
}
public void testTypeCheckBasicDowncast() throws Exception {
testTypes("/** @constructor */function foo() {}\n" +
"/** @type {Object} */ var bar = new foo();\n");
}
public void testTypeCheckNoDowncastToNumber() throws Exception {
testTypes("/** @constructor */function foo() {}\n" +
"/** @type {!Number} */ var bar = new foo();\n",
"initializing variable\n" +
"found : foo\n" +
"required: Number");
}
public void testTypeCheck21() throws Exception {
testTypes("/** @type Array.<String> */var foo;");
}
public void testTypeCheck22() throws Exception {
testTypes("/** @param {Element|Object} p */\nfunction foo(p){}\n" +
"/** @constructor */function Element(){}\n" +
"/** @type {Element|Object} */var v;\n" +
"foo(v);\n");
}
public void testTypeCheck23() throws Exception {
testTypes("/** @type {(Object,Null)} */var foo; foo = null;");
}
public void testTypeCheck24() throws Exception {
testTypes("/** @constructor */function MyType(){}\n" +
"/** @type {(MyType,Null)} */var foo; foo = null;");
}
public void testTypeCheckDefaultExterns() throws Exception {
testTypes("/** @param {string} x */ function f(x) {}" +
"f([].length);" ,
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testTypeCheckCustomExterns() throws Exception {
testTypes(
DEFAULT_EXTERNS + "/** @type {boolean} */ Array.prototype.oogabooga;",
"/** @param {string} x */ function f(x) {}" +
"f([].oogabooga);" ,
"actual parameter 1 of f does not match formal parameter\n" +
"found : boolean\n" +
"required: string", false);
}
public void testTypeCheckCustomExterns2() throws Exception {
testTypes(
DEFAULT_EXTERNS + "/** @enum {string} */ var Enum = {FOO: 1, BAR: 1};",
"/** @param {Enum} x */ function f(x) {} f(Enum.FOO); f(true);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : boolean\n" +
"required: Enum.<string>",
false);
}
public void testParameterizedArray1() throws Exception {
testTypes("/** @param {!Array.<number>} a\n" +
"* @return {string}\n" +
"*/ var f = function(a) { return a[0]; };",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testParameterizedArray2() throws Exception {
testTypes("/** @param {!Array.<!Array.<number>>} a\n" +
"* @return {number}\n" +
"*/ var f = function(a) { return a[0]; };",
"inconsistent return type\n" +
"found : Array.<number>\n" +
"required: number");
}
public void testParameterizedArray3() throws Exception {
testTypes("/** @param {!Array.<number>} a\n" +
"* @return {number}\n" +
"*/ var f = function(a) { a[1] = 0; return a[0]; };");
}
public void testParameterizedArray4() throws Exception {
testTypes("/** @param {!Array.<number>} a\n" +
"*/ var f = function(a) { a[0] = 'a'; };",
"assignment\n" +
"found : string\n" +
"required: number");
}
public void testParameterizedArray5() throws Exception {
testTypes("/** @param {!Array.<*>} a\n" +
"*/ var f = function(a) { a[0] = 'a'; };");
}
public void testParameterizedArray6() throws Exception {
testTypes("/** @param {!Array.<*>} a\n" +
"* @return {string}\n" +
"*/ var f = function(a) { return a[0]; };",
"inconsistent return type\n" +
"found : *\n" +
"required: string");
}
public void testParameterizedArray7() throws Exception {
testTypes("/** @param {?Array.<number>} a\n" +
"* @return {string}\n" +
"*/ var f = function(a) { return a[0]; };",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testParameterizedObject1() throws Exception {
testTypes("/** @param {!Object.<number>} a\n" +
"* @return {string}\n" +
"*/ var f = function(a) { return a[0]; };",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testParameterizedObject2() throws Exception {
testTypes("/** @param {!Object.<string,number>} a\n" +
"* @return {string}\n" +
"*/ var f = function(a) { return a['x']; };",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testParameterizedObject3() throws Exception {
testTypes("/** @param {!Object.<number,string>} a\n" +
"* @return {string}\n" +
"*/ var f = function(a) { return a['x']; };",
"restricted index type\n" +
"found : string\n" +
"required: number");
}
public void testParameterizedObject4() throws Exception {
testTypes("/** @enum {string} */ var E = {A: 'a', B: 'b'};\n" +
"/** @param {!Object.<E,string>} a\n" +
"* @return {string}\n" +
"*/ var f = function(a) { return a['x']; };",
"restricted index type\n" +
"found : string\n" +
"required: E.<string>");
}
public void testParameterizedObject5() throws Exception {
testTypes("/** @constructor */ function F() {" +
" /** @type {Object.<number, string>} */ this.numbers = {};" +
"}" +
"(new F()).numbers['ten'] = '10';",
"restricted index type\n" +
"found : string\n" +
"required: number");
}
public void testUnionOfFunctionAndType() throws Exception {
testTypes("/** @type {null|(function(Number):void)} */ var a;" +
"/** @type {(function(Number):void)|null} */ var b = null; a = b;");
}
public void testOptionalParameterComparedToUndefined() throws Exception {
testTypes("/**@param opt_a {Number}*/function foo(opt_a)" +
"{if (opt_a==undefined) var b = 3;}");
}
public void testOptionalAllType() throws Exception {
testTypes("/** @param {*} opt_x */function f(opt_x) { return opt_x }\n" +
"/** @type {*} */var y;\n" +
"f(y);");
}
public void testOptionalUnknownNamedType() throws Exception {
testTypes("/** @param {!T} opt_x\n@return {undefined} */\n" +
"function f(opt_x) { return opt_x; }\n" +
"/** @constructor */var T = function() {};",
"inconsistent return type\n" +
"found : (T|undefined)\n" +
"required: undefined");
}
public void testOptionalArgFunctionParam() throws Exception {
testTypes("/** @param {function(number=)} a */" +
"function f(a) {a()};");
}
public void testOptionalArgFunctionParam2() throws Exception {
testTypes("/** @param {function(number=)} a */" +
"function f(a) {a(3)};");
}
public void testOptionalArgFunctionParam3() throws Exception {
testTypes("/** @param {function(number=)} a */" +
"function f(a) {a(undefined)};");
}
public void testOptionalArgFunctionParam4() throws Exception {
String expectedWarning = "Function a: called with 2 argument(s). " +
"Function requires at least 0 argument(s) and no more than 1 " +
"argument(s).";
testTypes("/** @param {function(number=)} a */function f(a) {a(3,4)};",
expectedWarning, false);
}
public void testOptionalArgFunctionParamError() throws Exception {
String expectedWarning =
"Bad type annotation. variable length argument must be last";
testTypes("/** @param {function(...[number], number=)} a */" +
"function f(a) {};", expectedWarning, false);
}
public void testOptionalNullableArgFunctionParam() throws Exception {
testTypes("/** @param {function(?number=)} a */" +
"function f(a) {a()};");
}
public void testOptionalNullableArgFunctionParam2() throws Exception {
testTypes("/** @param {function(?number=)} a */" +
"function f(a) {a(null)};");
}
public void testOptionalNullableArgFunctionParam3() throws Exception {
testTypes("/** @param {function(?number=)} a */" +
"function f(a) {a(3)};");
}
public void testOptionalArgFunctionReturn() throws Exception {
testTypes("/** @return {function(number=)} */" +
"function f() { return function(opt_x) { }; };" +
"f()()");
}
public void testOptionalArgFunctionReturn2() throws Exception {
testTypes("/** @return {function(Object=)} */" +
"function f() { return function(opt_x) { }; };" +
"f()({})");
}
public void testBooleanType() throws Exception {
testTypes("/**@type {boolean} */var x = 1 < 2;");
}
public void testBooleanReduction1() throws Exception {
testTypes("/**@type {string} */var x; x = null || \"a\";");
}
public void testBooleanReduction2() throws Exception {
// It's important for the type system to recognize that in no case
// can the boolean expression evaluate to a boolean value.
testTypes("/** @param {string} s\n @return {string} */" +
"(function(s) { return ((s == 'a') && s) || 'b'; })");
}
public void testBooleanReduction3() throws Exception {
testTypes("/** @param {string} s\n @return {string?} */" +
"(function(s) { return s && null && 3; })");
}
public void testBooleanReduction4() throws Exception {
testTypes("/** @param {Object} x\n @return {Object} */" +
"(function(x) { return null || x || null ; })");
}
public void testBooleanReduction5() throws Exception {
testTypes("/**\n" +
"* @param {Array|string} x\n" +
"* @return {string?}\n" +
"*/\n" +
"var f = function(x) {\n" +
"if (!x || typeof x == 'string') {\n" +
"return x;\n" +
"}\n" +
"return null;\n" +
"};");
}
public void testBooleanReduction6() throws Exception {
testTypes("/**\n" +
"* @param {Array|string|null} x\n" +
"* @return {string?}\n" +
"*/\n" +
"var f = function(x) {\n" +
"if (!(x && typeof x != 'string')) {\n" +
"return x;\n" +
"}\n" +
"return null;\n" +
"};");
}
public void testBooleanReduction7() throws Exception {
testTypes("/** @constructor */var T = function() {};\n" +
"/**\n" +
"* @param {Array|T} x\n" +
"* @return {null}\n" +
"*/\n" +
"var f = function(x) {\n" +
"if (!x) {\n" +
"return x;\n" +
"}\n" +
"return null;\n" +
"};");
}
public void testNullAnd() throws Exception {
testTypes("/** @type null */var x;\n" +
"/** @type number */var r = x && x;",
"initializing variable\n" +
"found : null\n" +
"required: number");
}
public void testNullOr() throws Exception {
testTypes("/** @type null */var x;\n" +
"/** @type number */var r = x || x;",
"initializing variable\n" +
"found : null\n" +
"required: number");
}
public void testBooleanPreservation1() throws Exception {
testTypes("/**@type {string} */var x = \"a\";" +
"x = ((x == \"a\") && x) || x == \"b\";",
"assignment\n" +
"found : (boolean|string)\n" +
"required: string");
}
public void testBooleanPreservation2() throws Exception {
testTypes("/**@type {string} */var x = \"a\"; x = (x == \"a\") || x;",
"assignment\n" +
"found : (boolean|string)\n" +
"required: string");
}
public void testBooleanPreservation3() throws Exception {
testTypes("/** @param {Function?} x\n @return {boolean?} */" +
"function f(x) { return x && x == \"a\"; }",
"condition always evaluates to false\n" +
"left : Function\n" +
"right: string");
}
public void testBooleanPreservation4() throws Exception {
testTypes("/** @param {Function?|boolean} x\n @return {boolean} */" +
"function f(x) { return x && x == \"a\"; }",
"inconsistent return type\n" +
"found : (boolean|null)\n" +
"required: boolean");
}
public void testTypeOfReduction1() throws Exception {
testTypes("/** @param {string|number} x\n @return {string} */ " +
"function f(x) { return typeof x == 'number' ? String(x) : x; }");
}
public void testTypeOfReduction2() throws Exception {
testTypes("/** @param {string|number} x\n @return {string} */ " +
"function f(x) { return typeof x != 'string' ? String(x) : x; }");
}
public void testTypeOfReduction3() throws Exception {
testTypes("/** @param {number|null} x\n @return {number} */ " +
"function f(x) { return typeof x == 'object' ? 1 : x; }");
}
public void testTypeOfReduction4() throws Exception {
testTypes("/** @param {Object|undefined} x\n @return {Object} */ " +
"function f(x) { return typeof x == 'undefined' ? {} : x; }");
}
public void testTypeOfReduction5() throws Exception {
testTypes("/** @enum {string} */ var E = {A: 'a', B: 'b'};\n" +
"/** @param {!E|number} x\n @return {string} */ " +
"function f(x) { return typeof x != 'number' ? x : 'a'; }");
}
public void testTypeOfReduction6() throws Exception {
testTypes("/** @param {number|string} x\n@return {string} */\n" +
"function f(x) {\n" +
"return typeof x == 'string' && x.length == 3 ? x : 'a';\n" +
"}");
}
public void testTypeOfReduction7() throws Exception {
testTypes("/** @return {string} */var f = function(x) { " +
"return typeof x == 'number' ? x : 'a'; }",
"inconsistent return type\n" +
"found : (number|string)\n" +
"required: string");
}
public void testTypeOfReduction8() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @param {number|string} x\n@return {string} */\n" +
"function f(x) {\n" +
"return goog.isString(x) && x.length == 3 ? x : 'a';\n" +
"}", null);
}
public void testTypeOfReduction9() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @param {!Array|string} x\n@return {string} */\n" +
"function f(x) {\n" +
"return goog.isArray(x) ? 'a' : x;\n" +
"}", null);
}
public void testTypeOfReduction10() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @param {Array|string} x\n@return {Array} */\n" +
"function f(x) {\n" +
"return goog.isArray(x) ? x : [];\n" +
"}", null);
}
public void testTypeOfReduction11() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @param {Array|string} x\n@return {Array} */\n" +
"function f(x) {\n" +
"return goog.isObject(x) ? x : [];\n" +
"}", null);
}
public void testTypeOfReduction12() throws Exception {
testTypes("/** @enum {string} */ var E = {A: 'a', B: 'b'};\n" +
"/** @param {E|Array} x\n @return {Array} */ " +
"function f(x) { return typeof x == 'object' ? x : []; }");
}
public void testTypeOfReduction13() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @enum {string} */ var E = {A: 'a', B: 'b'};\n" +
"/** @param {E|Array} x\n@return {Array} */ " +
"function f(x) { return goog.isObject(x) ? x : []; }", null);
}
public void testTypeOfReduction14() throws Exception {
// Don't do type inference on GETELEMs.
testClosureTypes(
CLOSURE_DEFS +
"function f(x) { " +
" return goog.isString(arguments[0]) ? arguments[0] : 0;" +
"}", null);
}
public void testTypeOfReduction15() throws Exception {
// Don't do type inference on GETELEMs.
testClosureTypes(
CLOSURE_DEFS +
"function f(x) { " +
" return typeof arguments[0] == 'string' ? arguments[0] : 0;" +
"}", null);
}
public void testQualifiedNameReduction1() throws Exception {
testTypes("var x = {}; /** @type {string?} */ x.a = 'a';\n" +
"/** @return {string} */ var f = function() {\n" +
"return x.a ? x.a : 'a'; }");
}
public void testQualifiedNameReduction2() throws Exception {
testTypes("/** @param {string?} a\n@constructor */ var T = " +
"function(a) {this.a = a};\n" +
"/** @return {string} */ T.prototype.f = function() {\n" +
"return this.a ? this.a : 'a'; }");
}
public void testQualifiedNameReduction3() throws Exception {
testTypes("/** @param {string|Array} a\n@constructor */ var T = " +
"function(a) {this.a = a};\n" +
"/** @return {string} */ T.prototype.f = function() {\n" +
"return typeof this.a == 'string' ? this.a : 'a'; }");
}
public void testQualifiedNameReduction4() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @param {string|Array} a\n@constructor */ var T = " +
"function(a) {this.a = a};\n" +
"/** @return {string} */ T.prototype.f = function() {\n" +
"return goog.isString(this.a) ? this.a : 'a'; }", null);
}
public void testQualifiedNameReduction5a() throws Exception {
testTypes("var x = {/** @type {string} */ a:'b' };\n" +
"/** @return {string} */ var f = function() {\n" +
"return x.a; }");
}
public void testQualifiedNameReduction5b() throws Exception {
testTypes(
"var x = {/** @type {number} */ a:12 };\n" +
"/** @return {string} */\n" +
"var f = function() {\n" +
" return x.a;\n" +
"}",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testQualifiedNameReduction5c() throws Exception {
testTypes(
"/** @return {string} */ var f = function() {\n" +
"var x = {/** @type {number} */ a:0 };\n" +
"return (x.a) ? (x.a) : 'a'; }",
"inconsistent return type\n" +
"found : (number|string)\n" +
"required: string");
}
public void testQualifiedNameReduction6() throws Exception {
testTypes(
"/** @return {string} */ var f = function() {\n" +
"var x = {/** @return {string?} */ get a() {return 'a'}};\n" +
"return x.a ? x.a : 'a'; }");
}
public void testQualifiedNameReduction7() throws Exception {
testTypes(
"/** @return {string} */ var f = function() {\n" +
"var x = {/** @return {number} */ get a() {return 12}};\n" +
"return x.a; }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testQualifiedNameReduction7a() throws Exception {
// It would be nice to find a way to make this an error.
testTypes(
"/** @return {string} */ var f = function() {\n" +
"var x = {get a() {return 12}};\n" +
"return x.a; }");
}
public void testQualifiedNameReduction8() throws Exception {
testTypes(
"/** @return {string} */ var f = function() {\n" +
"var x = {get a() {return 'a'}};\n" +
"return x.a ? x.a : 'a'; }");
}
public void testQualifiedNameReduction9() throws Exception {
testTypes(
"/** @return {string} */ var f = function() {\n" +
"var x = { /** @param {string} b */ set a(b) {}};\n" +
"return x.a ? x.a : 'a'; }");
}
public void testQualifiedNameReduction10() throws Exception {
// TODO(johnlenz): separate setter property types from getter property
// types.
testTypes(
"/** @return {string} */ var f = function() {\n" +
"var x = { /** @param {number} b */ set a(b) {}};\n" +
"return x.a ? x.a : 'a'; }",
"inconsistent return type\n" +
"found : (number|string)\n" +
"required: string");
}
public void testObjLitDef1a() throws Exception {
testTypes(
"var x = {/** @type {number} */ a:12 };\n" +
"x.a = 'a';",
"assignment to property a of x\n" +
"found : string\n" +
"required: number");
}
public void testObjLitDef1b() throws Exception {
testTypes(
"function f(){" +
"var x = {/** @type {number} */ a:12 };\n" +
"x.a = 'a';" +
"};\n" +
"f();",
"assignment to property a of x\n" +
"found : string\n" +
"required: number");
}
public void testObjLitDef2a() throws Exception {
testTypes(
"var x = {/** @param {number} b */ set a(b){} };\n" +
"x.a = 'a';",
"assignment to property a of x\n" +
"found : string\n" +
"required: number");
}
public void testObjLitDef2b() throws Exception {
testTypes(
"function f(){" +
"var x = {/** @param {number} b */ set a(b){} };\n" +
"x.a = 'a';" +
"};\n" +
"f();",
"assignment to property a of x\n" +
"found : string\n" +
"required: number");
}
public void testObjLitDef3a() throws Exception {
testTypes(
"/** @type {string} */ var y;\n" +
"var x = {/** @return {number} */ get a(){} };\n" +
"y = x.a;",
"assignment\n" +
"found : number\n" +
"required: string");
}
public void testObjLitDef3b() throws Exception {
testTypes(
"/** @type {string} */ var y;\n" +
"function f(){" +
"var x = {/** @return {number} */ get a(){} };\n" +
"y = x.a;" +
"};\n" +
"f();",
"assignment\n" +
"found : number\n" +
"required: string");
}
public void testObjLitDef4() throws Exception {
testTypes(
"var x = {" +
"/** @return {number} */ a:12 };\n",
"assignment to property a of {a: function (): number}\n" +
"found : number\n" +
"required: function (): number");
}
public void testObjLitDef5() throws Exception {
testTypes(
"var x = {};\n" +
"/** @return {number} */ x.a = 12;\n",
"assignment to property a of x\n" +
"found : number\n" +
"required: function (): number");
}
public void testInstanceOfReduction1() throws Exception {
testTypes("/** @constructor */ var T = function() {};\n" +
"/** @param {T|string} x\n@return {T} */\n" +
"var f = function(x) {\n" +
"if (x instanceof T) { return x; } else { return new T(); }\n" +
"};");
}
public void testInstanceOfReduction2() throws Exception {
testTypes("/** @constructor */ var T = function() {};\n" +
"/** @param {!T|string} x\n@return {string} */\n" +
"var f = function(x) {\n" +
"if (x instanceof T) { return ''; } else { return x; }\n" +
"};");
}
public void testUndeclaredGlobalProperty1() throws Exception {
testTypes("/** @const */ var x = {}; x.y = null;" +
"function f(a) { x.y = a; }" +
"/** @param {string} a */ function g(a) { }" +
"function h() { g(x.y); }");
}
public void testUndeclaredGlobalProperty2() throws Exception {
testTypes("/** @const */ var x = {}; x.y = null;" +
"function f() { x.y = 3; }" +
"/** @param {string} a */ function g(a) { }" +
"function h() { g(x.y); }",
"actual parameter 1 of g does not match formal parameter\n" +
"found : (null|number)\n" +
"required: string");
}
public void testLocallyInferredGlobalProperty1() throws Exception {
// We used to have a bug where x.y.z leaked from f into h.
testTypes(
"/** @constructor */ function F() {}" +
"/** @type {number} */ F.prototype.z;" +
"/** @const */ var x = {}; /** @type {F} */ x.y;" +
"function f() { x.y.z = 'abc'; }" +
"/** @param {number} x */ function g(x) {}" +
"function h() { g(x.y.z); }",
"assignment to property z of F\n" +
"found : string\n" +
"required: number");
}
public void testPropertyInferredPropagation() throws Exception {
testTypes("/** @return {Object} */function f() { return {}; }\n" +
"function g() { var x = f(); if (x.p) x.a = 'a'; else x.a = 'b'; }\n" +
"function h() { var x = f(); x.a = false; }");
}
public void testPropertyInference1() throws Exception {
testTypes(
"/** @constructor */ function F() { this.x_ = true; }" +
"/** @return {string} */" +
"F.prototype.bar = function() { if (this.x_) return this.x_; };",
"inconsistent return type\n" +
"found : boolean\n" +
"required: string");
}
public void testPropertyInference2() throws Exception {
testTypes(
"/** @constructor */ function F() { this.x_ = true; }" +
"F.prototype.baz = function() { this.x_ = null; };" +
"/** @return {string} */" +
"F.prototype.bar = function() { if (this.x_) return this.x_; };",
"inconsistent return type\n" +
"found : boolean\n" +
"required: string");
}
public void testPropertyInference3() throws Exception {
testTypes(
"/** @constructor */ function F() { this.x_ = true; }" +
"F.prototype.baz = function() { this.x_ = 3; };" +
"/** @return {string} */" +
"F.prototype.bar = function() { if (this.x_) return this.x_; };",
"inconsistent return type\n" +
"found : (boolean|number)\n" +
"required: string");
}
public void testPropertyInference4() throws Exception {
testTypes(
"/** @constructor */ function F() { }" +
"F.prototype.x_ = 3;" +
"/** @return {string} */" +
"F.prototype.bar = function() { if (this.x_) return this.x_; };",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testPropertyInference5() throws Exception {
testTypes(
"/** @constructor */ function F() { }" +
"F.prototype.baz = function() { this.x_ = 3; };" +
"/** @return {string} */" +
"F.prototype.bar = function() { if (this.x_) return this.x_; };");
}
public void testPropertyInference6() throws Exception {
testTypes(
"/** @constructor */ function F() { }" +
"(new F).x_ = 3;" +
"/** @return {string} */" +
"F.prototype.bar = function() { return this.x_; };");
}
public void testPropertyInference7() throws Exception {
testTypes(
"/** @constructor */ function F() { this.x_ = true; }" +
"(new F).x_ = 3;" +
"/** @return {string} */" +
"F.prototype.bar = function() { return this.x_; };",
"inconsistent return type\n" +
"found : boolean\n" +
"required: string");
}
public void testPropertyInference8() throws Exception {
testTypes(
"/** @constructor */ function F() { " +
" /** @type {string} */ this.x_ = 'x';" +
"}" +
"(new F).x_ = 3;" +
"/** @return {string} */" +
"F.prototype.bar = function() { return this.x_; };",
"assignment to property x_ of F\n" +
"found : number\n" +
"required: string");
}
public void testPropertyInference9() throws Exception {
testTypes(
"/** @constructor */ function A() {}" +
"/** @return {function(): ?} */ function f() { " +
" return function() {};" +
"}" +
"var g = f();" +
"/** @type {number} */ g.prototype.bar_ = null;",
"assignment\n" +
"found : null\n" +
"required: number");
}
public void testPropertyInference10() throws Exception {
// NOTE(nicksantos): There used to be a bug where a property
// on the prototype of one structural function would leak onto
// the prototype of other variables with the same structural
// function type.
testTypes(
"/** @constructor */ function A() {}" +
"/** @return {function(): ?} */ function f() { " +
" return function() {};" +
"}" +
"var g = f();" +
"/** @type {number} */ g.prototype.bar_ = 1;" +
"var h = f();" +
"/** @type {string} */ h.prototype.bar_ = 1;",
"assignment\n" +
"found : number\n" +
"required: string");
}
public void testNoPersistentTypeInferenceForObjectProperties()
throws Exception {
testTypes("/** @param {Object} o\n@param {string} x */\n" +
"function s1(o,x) { o.x = x; }\n" +
"/** @param {Object} o\n@return {string} */\n" +
"function g1(o) { return typeof o.x == 'undefined' ? '' : o.x; }\n" +
"/** @param {Object} o\n@param {number} x */\n" +
"function s2(o,x) { o.x = x; }\n" +
"/** @param {Object} o\n@return {number} */\n" +
"function g2(o) { return typeof o.x == 'undefined' ? 0 : o.x; }");
}
public void testNoPersistentTypeInferenceForFunctionProperties()
throws Exception {
testTypes("/** @param {Function} o\n@param {string} x */\n" +
"function s1(o,x) { o.x = x; }\n" +
"/** @param {Function} o\n@return {string} */\n" +
"function g1(o) { return typeof o.x == 'undefined' ? '' : o.x; }\n" +
"/** @param {Function} o\n@param {number} x */\n" +
"function s2(o,x) { o.x = x; }\n" +
"/** @param {Function} o\n@return {number} */\n" +
"function g2(o) { return typeof o.x == 'undefined' ? 0 : o.x; }");
}
public void testObjectPropertyTypeInferredInLocalScope1() throws Exception {
testTypes("/** @param {!Object} o\n@return {string} */\n" +
"function f(o) { o.x = 1; return o.x; }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testObjectPropertyTypeInferredInLocalScope2() throws Exception {
testTypes("/**@param {!Object} o\n@param {number?} x\n@return {string}*/" +
"function f(o, x) { o.x = 'a';\nif (x) {o.x = x;}\nreturn o.x; }",
"inconsistent return type\n" +
"found : (number|string)\n" +
"required: string");
}
public void testObjectPropertyTypeInferredInLocalScope3() throws Exception {
testTypes("/**@param {!Object} o\n@param {number?} x\n@return {string}*/" +
"function f(o, x) { if (x) {o.x = x;} else {o.x = 'a';}\nreturn o.x; }",
"inconsistent return type\n" +
"found : (number|string)\n" +
"required: string");
}
public void testMismatchingOverridingInferredPropertyBeforeDeclaredProperty1()
throws Exception {
testTypes("/** @constructor */var T = function() { this.x = ''; };\n" +
"/** @type {number} */ T.prototype.x = 0;",
"assignment to property x of T\n" +
"found : string\n" +
"required: number");
}
public void testMismatchingOverridingInferredPropertyBeforeDeclaredProperty2()
throws Exception {
testTypes("/** @constructor */var T = function() { this.x = ''; };\n" +
"/** @type {number} */ T.prototype.x;",
"assignment to property x of T\n" +
"found : string\n" +
"required: number");
}
public void testMismatchingOverridingInferredPropertyBeforeDeclaredProperty3()
throws Exception {
testTypes("/** @type {Object} */ var n = {};\n" +
"/** @constructor */ n.T = function() { this.x = ''; };\n" +
"/** @type {number} */ n.T.prototype.x = 0;",
"assignment to property x of n.T\n" +
"found : string\n" +
"required: number");
}
public void testMismatchingOverridingInferredPropertyBeforeDeclaredProperty4()
throws Exception {
testTypes("var n = {};\n" +
"/** @constructor */ n.T = function() { this.x = ''; };\n" +
"/** @type {number} */ n.T.prototype.x = 0;",
"assignment to property x of n.T\n" +
"found : string\n" +
"required: number");
}
public void testPropertyUsedBeforeDefinition1() throws Exception {
testTypes("/** @constructor */ var T = function() {};\n" +
"/** @return {string} */" +
"T.prototype.f = function() { return this.g(); };\n" +
"/** @return {number} */ T.prototype.g = function() { return 1; };\n",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testPropertyUsedBeforeDefinition2() throws Exception {
testTypes("var n = {};\n" +
"/** @constructor */ n.T = function() {};\n" +
"/** @return {string} */" +
"n.T.prototype.f = function() { return this.g(); };\n" +
"/** @return {number} */ n.T.prototype.g = function() { return 1; };\n",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testAdd1() throws Exception {
testTypes("/**@return {void}*/function foo(){var a = 'abc'+foo();}");
}
public void testAdd2() throws Exception {
testTypes("/**@return {void}*/function foo(){var a = foo()+4;}");
}
public void testAdd3() throws Exception {
testTypes("/** @type {string} */ var a = 'a';" +
"/** @type {string} */ var b = 'b';" +
"/** @type {string} */ var c = a + b;");
}
public void testAdd4() throws Exception {
testTypes("/** @type {number} */ var a = 5;" +
"/** @type {string} */ var b = 'b';" +
"/** @type {string} */ var c = a + b;");
}
public void testAdd5() throws Exception {
testTypes("/** @type {string} */ var a = 'a';" +
"/** @type {number} */ var b = 5;" +
"/** @type {string} */ var c = a + b;");
}
public void testAdd6() throws Exception {
testTypes("/** @type {number} */ var a = 5;" +
"/** @type {number} */ var b = 5;" +
"/** @type {number} */ var c = a + b;");
}
public void testAdd7() throws Exception {
testTypes("/** @type {number} */ var a = 5;" +
"/** @type {string} */ var b = 'b';" +
"/** @type {number} */ var c = a + b;",
"initializing variable\n" +
"found : string\n" +
"required: number");
}
public void testAdd8() throws Exception {
testTypes("/** @type {string} */ var a = 'a';" +
"/** @type {number} */ var b = 5;" +
"/** @type {number} */ var c = a + b;",
"initializing variable\n" +
"found : string\n" +
"required: number");
}
public void testAdd9() throws Exception {
testTypes("/** @type {number} */ var a = 5;" +
"/** @type {number} */ var b = 5;" +
"/** @type {string} */ var c = a + b;",
"initializing variable\n" +
"found : number\n" +
"required: string");
}
public void testAdd10() throws Exception {
// d.e.f will have unknown type.
testTypes(
suppressMissingProperty("e", "f") +
"/** @type {number} */ var a = 5;" +
"/** @type {string} */ var c = a + d.e.f;");
}
public void testAdd11() throws Exception {
// d.e.f will have unknown type.
testTypes(
suppressMissingProperty("e", "f") +
"/** @type {number} */ var a = 5;" +
"/** @type {number} */ var c = a + d.e.f;");
}
public void testAdd12() throws Exception {
testTypes("/** @return {(number,string)} */ function a() { return 5; }" +
"/** @type {number} */ var b = 5;" +
"/** @type {boolean} */ var c = a() + b;",
"initializing variable\n" +
"found : (number|string)\n" +
"required: boolean");
}
public void testAdd13() throws Exception {
testTypes("/** @type {number} */ var a = 5;" +
"/** @return {(number,string)} */ function b() { return 5; }" +
"/** @type {boolean} */ var c = a + b();",
"initializing variable\n" +
"found : (number|string)\n" +
"required: boolean");
}
public void testAdd14() throws Exception {
testTypes("/** @type {(null,string)} */ var a = null;" +
"/** @type {number} */ var b = 5;" +
"/** @type {boolean} */ var c = a + b;",
"initializing variable\n" +
"found : (number|string)\n" +
"required: boolean");
}
public void testAdd15() throws Exception {
testTypes("/** @type {number} */ var a = 5;" +
"/** @return {(number,string)} */ function b() { return 5; }" +
"/** @type {boolean} */ var c = a + b();",
"initializing variable\n" +
"found : (number|string)\n" +
"required: boolean");
}
public void testAdd16() throws Exception {
testTypes("/** @type {(undefined,string)} */ var a = undefined;" +
"/** @type {number} */ var b = 5;" +
"/** @type {boolean} */ var c = a + b;",
"initializing variable\n" +
"found : (number|string)\n" +
"required: boolean");
}
public void testAdd17() throws Exception {
testTypes("/** @type {number} */ var a = 5;" +
"/** @type {(undefined,string)} */ var b = undefined;" +
"/** @type {boolean} */ var c = a + b;",
"initializing variable\n" +
"found : (number|string)\n" +
"required: boolean");
}
public void testAdd18() throws Exception {
testTypes("function f() {};" +
"/** @type {string} */ var a = 'a';" +
"/** @type {number} */ var c = a + f();",
"initializing variable\n" +
"found : string\n" +
"required: number");
}
public void testAdd19() throws Exception {
testTypes("/** @param {number} opt_x\n@param {number} opt_y\n" +
"@return {number} */ function f(opt_x, opt_y) {" +
"return opt_x + opt_y;}");
}
public void testAdd20() throws Exception {
testTypes("/** @param {!Number} opt_x\n@param {!Number} opt_y\n" +
"@return {number} */ function f(opt_x, opt_y) {" +
"return opt_x + opt_y;}");
}
public void testAdd21() throws Exception {
testTypes("/** @param {Number|Boolean} opt_x\n" +
"@param {number|boolean} opt_y\n" +
"@return {number} */ function f(opt_x, opt_y) {" +
"return opt_x + opt_y;}");
}
public void testNumericComparison1() throws Exception {
testTypes("/**@param {number} a*/ function f(a) {return a < 3;}");
}
public void testNumericComparison2() throws Exception {
testTypes("/**@param {!Object} a*/ function f(a) {return a < 3;}",
"left side of numeric comparison\n" +
"found : Object\n" +
"required: number");
}
public void testNumericComparison3() throws Exception {
testTypes("/**@param {string} a*/ function f(a) {return a < 3;}");
}
public void testNumericComparison4() throws Exception {
testTypes("/**@param {(number,undefined)} a*/ " +
"function f(a) {return a < 3;}");
}
public void testNumericComparison5() throws Exception {
testTypes("/**@param {*} a*/ function f(a) {return a < 3;}",
"left side of numeric comparison\n" +
"found : *\n" +
"required: number");
}
public void testNumericComparison6() throws Exception {
testTypes("/**@return {void} */ function foo() { if (3 >= foo()) return; }",
"right side of numeric comparison\n" +
"found : undefined\n" +
"required: number");
}
public void testStringComparison1() throws Exception {
testTypes("/**@param {string} a*/ function f(a) {return a < 'x';}");
}
public void testStringComparison2() throws Exception {
testTypes("/**@param {Object} a*/ function f(a) {return a < 'x';}");
}
public void testStringComparison3() throws Exception {
testTypes("/**@param {number} a*/ function f(a) {return a < 'x';}");
}
public void testStringComparison4() throws Exception {
testTypes("/**@param {string|undefined} a*/ " +
"function f(a) {return a < 'x';}");
}
public void testStringComparison5() throws Exception {
testTypes("/**@param {*} a*/ " +
"function f(a) {return a < 'x';}");
}
public void testStringComparison6() throws Exception {
testTypes("/**@return {void} */ " +
"function foo() { if ('a' >= foo()) return; }",
"right side of comparison\n" +
"found : undefined\n" +
"required: string");
}
public void testValueOfComparison1() throws Exception {
testTypes("/** @constructor */function O() {};" +
"/**@override*/O.prototype.valueOf = function() { return 1; };" +
"/**@param {!O} a\n@param {!O} b*/ function f(a,b) { return a < b; }");
}
public void testValueOfComparison2() throws Exception {
testTypes("/** @constructor */function O() {};" +
"/**@override*/O.prototype.valueOf = function() { return 1; };" +
"/**@param {!O} a\n@param {number} b*/" +
"function f(a,b) { return a < b; }");
}
public void testValueOfComparison3() throws Exception {
testTypes("/** @constructor */function O() {};" +
"/**@override*/O.prototype.toString = function() { return 'o'; };" +
"/**@param {!O} a\n@param {string} b*/" +
"function f(a,b) { return a < b; }");
}
public void testGenericRelationalExpression() throws Exception {
testTypes("/**@param {*} a\n@param {*} b*/ " +
"function f(a,b) {return a < b;}");
}
public void testInstanceof1() throws Exception {
testTypes("function foo(){" +
"if (bar instanceof 3)return;}",
"instanceof requires an object\n" +
"found : number\n" +
"required: Object");
}
public void testInstanceof2() throws Exception {
testTypes("/**@return {void}*/function foo(){" +
"if (foo() instanceof Object)return;}",
"deterministic instanceof yields false\n" +
"found : undefined\n" +
"required: NoObject");
}
public void testInstanceof3() throws Exception {
testTypes("/**@return {*} */function foo(){" +
"if (foo() instanceof Object)return;}");
}
public void testInstanceof4() throws Exception {
testTypes("/**@return {(Object|number)} */function foo(){" +
"if (foo() instanceof Object)return 3;}");
}
public void testInstanceof5() throws Exception {
// No warning for unknown types.
testTypes("/** @return {?} */ function foo(){" +
"if (foo() instanceof Object)return;}");
}
public void testInstanceof6() throws Exception {
testTypes("/**@return {(Array|number)} */function foo(){" +
"if (foo() instanceof Object)return 3;}");
}
public void testInstanceOfReduction3() throws Exception {
testTypes(
"/** \n" +
" * @param {Object} x \n" +
" * @param {Function} y \n" +
" * @return {boolean} \n" +
" */\n" +
"var f = function(x, y) {\n" +
" return x instanceof y;\n" +
"};");
}
public void testScoping1() throws Exception {
testTypes(
"/**@param {string} a*/function foo(a){" +
" /**@param {Array|string} a*/function bar(a){" +
" if (a instanceof Array)return;" +
" }" +
"}");
}
public void testScoping2() throws Exception {
testTypes(
"/** @type number */ var a;" +
"function Foo() {" +
" /** @type string */ var a;" +
"}");
}
public void testScoping3() throws Exception {
testTypes("\n\n/** @type{Number}*/var b;\n/** @type{!String} */var b;",
"variable b redefined with type String, original " +
"definition at [testcode]:3 with type (Number|null)");
}
public void testScoping4() throws Exception {
testTypes("/** @type{Number}*/var b; if (true) /** @type{!String} */var b;",
"variable b redefined with type String, original " +
"definition at [testcode]:1 with type (Number|null)");
}
public void testScoping5() throws Exception {
// multiple definitions are not checked by the type checker but by a
// subsequent pass
testTypes("if (true) var b; var b;");
}
public void testScoping6() throws Exception {
// multiple definitions are not checked by the type checker but by a
// subsequent pass
testTypes("if (true) var b; if (true) var b;");
}
public void testScoping7() throws Exception {
testTypes("/** @constructor */function A() {" +
" /** @type !A */this.a = null;" +
"}",
"assignment to property a of A\n" +
"found : null\n" +
"required: A");
}
public void testScoping8() throws Exception {
testTypes("/** @constructor */function A() {}" +
"/** @constructor */function B() {" +
" /** @type !A */this.a = null;" +
"}",
"assignment to property a of B\n" +
"found : null\n" +
"required: A");
}
public void testScoping9() throws Exception {
testTypes("/** @constructor */function B() {" +
" /** @type !A */this.a = null;" +
"}" +
"/** @constructor */function A() {}",
"assignment to property a of B\n" +
"found : null\n" +
"required: A");
}
public void testScoping10() throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope("var a = function b(){};");
// a declared, b is not
assertTrue(p.scope.isDeclared("a", false));
assertFalse(p.scope.isDeclared("b", false));
// checking that a has the correct assigned type
assertEquals("function (): undefined",
p.scope.getVar("a").getType().toString());
}
public void testScoping11() throws Exception {
// named function expressions create a binding in their body only
// the return is wrong but the assignment is ok since the type of b is ?
testTypes(
"/** @return {number} */var a = function b(){ return b };",
"inconsistent return type\n" +
"found : function (): number\n" +
"required: number");
}
public void testScoping12() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** @type {number} */ F.prototype.bar = 3;" +
"/** @param {!F} f */ function g(f) {" +
" /** @return {string} */" +
" function h() {" +
" return f.bar;" +
" }" +
"}",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testFunctionArguments1() throws Exception {
testFunctionType(
"/** @param {number} a\n@return {string} */" +
"function f(a) {}",
"function (number): string");
}
public void testFunctionArguments2() throws Exception {
testFunctionType(
"/** @param {number} opt_a\n@return {string} */" +
"function f(opt_a) {}",
"function (number=): string");
}
public void testFunctionArguments3() throws Exception {
testFunctionType(
"/** @param {number} b\n@return {string} */" +
"function f(a,b) {}",
"function (?, number): string");
}
public void testFunctionArguments4() throws Exception {
testFunctionType(
"/** @param {number} opt_a\n@return {string} */" +
"function f(a,opt_a) {}",
"function (?, number=): string");
}
public void testFunctionArguments5() throws Exception {
testTypes(
"function a(opt_a,a) {}",
"optional arguments must be at the end");
}
public void testFunctionArguments6() throws Exception {
testTypes(
"function a(var_args,a) {}",
"variable length argument must be last");
}
public void testFunctionArguments7() throws Exception {
testTypes(
"/** @param {number} opt_a\n@return {string} */" +
"function a(a,opt_a,var_args) {}");
}
public void testFunctionArguments8() throws Exception {
testTypes(
"function a(a,opt_a,var_args,b) {}",
"variable length argument must be last");
}
public void testFunctionArguments9() throws Exception {
// testing that only one error is reported
testTypes(
"function a(a,opt_a,var_args,b,c) {}",
"variable length argument must be last");
}
public void testFunctionArguments10() throws Exception {
// testing that only one error is reported
testTypes(
"function a(a,opt_a,b,c) {}",
"optional arguments must be at the end");
}
public void testFunctionArguments11() throws Exception {
testTypes(
"function a(a,opt_a,b,c,var_args,d) {}",
"optional arguments must be at the end");
}
public void testFunctionArguments12() throws Exception {
testTypes("/** @param foo {String} */function bar(baz){}",
"parameter foo does not appear in bar's parameter list");
}
public void testFunctionArguments13() throws Exception {
// verifying that the argument type have non-inferrable types
testTypes(
"/** @return {boolean} */ function u() { return true; }" +
"/** @param {boolean} b\n@return {?boolean} */" +
"function f(b) { if (u()) { b = null; } return b; }",
"assignment\n" +
"found : null\n" +
"required: boolean");
}
public void testFunctionArguments14() throws Exception {
testTypes(
"/**\n" +
" * @param {string} x\n" +
" * @param {number} opt_y\n" +
" * @param {boolean} var_args\n" +
" */ function f(x, opt_y, var_args) {}" +
"f('3'); f('3', 2); f('3', 2, true); f('3', 2, true, false);");
}
public void testFunctionArguments15() throws Exception {
testTypes(
"/** @param {?function(*)} f */" +
"function g(f) { f(1, 2); }",
"Function f: called with 2 argument(s). " +
"Function requires at least 1 argument(s) " +
"and no more than 1 argument(s).");
}
public void testFunctionArguments16() throws Exception {
testTypes(
"/** @param {...number} var_args */" +
"function g(var_args) {} g(1, true);",
"actual parameter 2 of g does not match formal parameter\n" +
"found : boolean\n" +
"required: (number|undefined)");
}
public void testPrintFunctionName1() throws Exception {
// Ensures that the function name is pretty.
testTypes(
"var goog = {}; goog.run = function(f) {};" +
"goog.run();",
"Function goog.run: called with 0 argument(s). " +
"Function requires at least 1 argument(s) " +
"and no more than 1 argument(s).");
}
public void testPrintFunctionName2() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {}; " +
"Foo.prototype.run = function(f) {};" +
"(new Foo).run();",
"Function Foo.prototype.run: called with 0 argument(s). " +
"Function requires at least 1 argument(s) " +
"and no more than 1 argument(s).");
}
public void testFunctionInference1() throws Exception {
testFunctionType(
"function f(a) {}",
"function (?): undefined");
}
public void testFunctionInference2() throws Exception {
testFunctionType(
"function f(a,b) {}",
"function (?, ?): undefined");
}
public void testFunctionInference3() throws Exception {
testFunctionType(
"function f(var_args) {}",
"function (...[?]): undefined");
}
public void testFunctionInference4() throws Exception {
testFunctionType(
"function f(a,b,c,var_args) {}",
"function (?, ?, ?, ...[?]): undefined");
}
public void testFunctionInference5() throws Exception {
testFunctionType(
"/** @this Date\n@return {string} */function f(a) {}",
"function (this:Date, ?): string");
}
public void testFunctionInference6() throws Exception {
testFunctionType(
"/** @this Date\n@return {string} */function f(opt_a) {}",
"function (this:Date, ?=): string");
}
public void testFunctionInference7() throws Exception {
testFunctionType(
"/** @this Date */function f(a,b,c,var_args) {}",
"function (this:Date, ?, ?, ?, ...[?]): undefined");
}
public void testFunctionInference8() throws Exception {
testFunctionType(
"function f() {}",
"function (): undefined");
}
public void testFunctionInference9() throws Exception {
testFunctionType(
"var f = function() {};",
"function (): undefined");
}
public void testFunctionInference10() throws Exception {
testFunctionType(
"/** @this Date\n@param {boolean} b\n@return {string} */" +
"var f = function(a,b) {};",
"function (this:Date, ?, boolean): string");
}
public void testFunctionInference11() throws Exception {
testFunctionType(
"var goog = {};" +
"/** @return {number}*/goog.f = function(){};",
"goog.f",
"function (): number");
}
public void testFunctionInference12() throws Exception {
testFunctionType(
"var goog = {};" +
"goog.f = function(){};",
"goog.f",
"function (): undefined");
}
public void testFunctionInference13() throws Exception {
testFunctionType(
"var goog = {};" +
"/** @constructor */ goog.Foo = function(){};" +
"/** @param {!goog.Foo} f */function eatFoo(f){};",
"eatFoo",
"function (goog.Foo): undefined");
}
public void testFunctionInference14() throws Exception {
testFunctionType(
"var goog = {};" +
"/** @constructor */ goog.Foo = function(){};" +
"/** @return {!goog.Foo} */function eatFoo(){ return new goog.Foo; };",
"eatFoo",
"function (): goog.Foo");
}
public void testFunctionInference15() throws Exception {
testFunctionType(
"/** @constructor */ function f() {};" +
"f.prototype.foo = function(){};",
"f.prototype.foo",
"function (this:f): undefined");
}
public void testFunctionInference16() throws Exception {
testFunctionType(
"/** @constructor */ function f() {};" +
"f.prototype.foo = function(){};",
"(new f).foo",
"function (this:f): undefined");
}
public void testFunctionInference17() throws Exception {
testFunctionType(
"/** @constructor */ function f() {}" +
"function abstractMethod() {}" +
"/** @param {number} x */ f.prototype.foo = abstractMethod;",
"(new f).foo",
"function (this:f, number): ?");
}
public void testFunctionInference18() throws Exception {
testFunctionType(
"var goog = {};" +
"/** @this {Date} */ goog.eatWithDate;",
"goog.eatWithDate",
"function (this:Date): ?");
}
public void testFunctionInference19() throws Exception {
testFunctionType(
"/** @param {string} x */ var f;",
"f",
"function (string): ?");
}
public void testFunctionInference20() throws Exception {
testFunctionType(
"/** @this {Date} */ var f;",
"f",
"function (this:Date): ?");
}
public void testInnerFunction1() throws Exception {
testTypes(
"function f() {" +
" /** @type {number} */ var x = 3;\n" +
" function g() { x = null; }" +
" return x;" +
"}",
"assignment\n" +
"found : null\n" +
"required: number");
}
public void testInnerFunction2() throws Exception {
testTypes(
"/** @return {number} */\n" +
"function f() {" +
" var x = null;\n" +
" function g() { x = 3; }" +
" g();" +
" return x;" +
"}",
"inconsistent return type\n" +
"found : (null|number)\n" +
"required: number");
}
public void testInnerFunction3() throws Exception {
testTypes(
"var x = null;" +
"/** @return {number} */\n" +
"function f() {" +
" x = 3;\n" +
" /** @return {number} */\n" +
" function g() { x = true; return x; }" +
" return x;" +
"}",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testInnerFunction4() throws Exception {
testTypes(
"var x = null;" +
"/** @return {number} */\n" +
"function f() {" +
" x = '3';\n" +
" /** @return {number} */\n" +
" function g() { x = 3; return x; }" +
" return x;" +
"}",
"inconsistent return type\n" +
"found : string\n" +
"required: number");
}
public void testInnerFunction5() throws Exception {
testTypes(
"/** @return {number} */\n" +
"function f() {" +
" var x = 3;\n" +
" /** @return {number} */" +
" function g() { var x = 3;x = true; return x; }" +
" return x;" +
"}",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testInnerFunction6() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"function f() {" +
" var x = 0 || function() {};\n" +
" function g() { if (goog.isFunction(x)) { x(1); } }" +
" g();" +
"}", null);
}
public void testInnerFunction7() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"function f() {" +
" /** @type {number|function()} */" +
" var x = 0 || function() {};\n" +
" function g() { if (goog.isFunction(x)) { x(1); } }" +
" g();" +
"}",
"Function x: called with 1 argument(s). " +
"Function requires at least 0 argument(s) " +
"and no more than 0 argument(s).");
}
public void testInnerFunction8() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"function f() {" +
" function x() {};\n" +
" function g() { if (goog.isFunction(x)) { x(1); } }" +
" g();" +
"}",
"Function x: called with 1 argument(s). " +
"Function requires at least 0 argument(s) " +
"and no more than 0 argument(s).");
}
public void testInnerFunction9() throws Exception {
testTypes(
"function f() {" +
" var x = 3;\n" +
" function g() { x = null; };\n" +
" function h() { return x == null; }" +
" return h();" +
"}");
}
public void testInnerFunction10() throws Exception {
testTypes(
"function f() {" +
" /** @type {?number} */ var x = null;" +
" /** @return {string} */" +
" function g() {" +
" if (!x) {" +
" x = 1;" +
" }" +
" return x;" +
" }" +
"}",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testInnerFunction11() throws Exception {
// TODO(nicksantos): This is actually bad inference, because
// h sets x to null. We should fix this, but for now we do it
// this way so that we don't break existing binaries. We will
// need to change TypeInference#isUnflowable to fix this.
testTypes(
"function f() {" +
" /** @type {?number} */ var x = null;" +
" /** @return {number} */" +
" function g() {" +
" x = 1;" +
" h();" +
" return x;" +
" }" +
" function h() {" +
" x = null;" +
" }" +
"}");
}
public void testAbstractMethodHandling1() throws Exception {
testTypes(
"/** @type {Function} */ var abstractFn = function() {};" +
"abstractFn(1);");
}
public void testAbstractMethodHandling2() throws Exception {
testTypes(
"var abstractFn = function() {};" +
"abstractFn(1);",
"Function abstractFn: called with 1 argument(s). " +
"Function requires at least 0 argument(s) " +
"and no more than 0 argument(s).");
}
public void testAbstractMethodHandling3() throws Exception {
testTypes(
"var goog = {};" +
"/** @type {Function} */ goog.abstractFn = function() {};" +
"goog.abstractFn(1);");
}
public void testAbstractMethodHandling4() throws Exception {
testTypes(
"var goog = {};" +
"goog.abstractFn = function() {};" +
"goog.abstractFn(1);",
"Function goog.abstractFn: called with 1 argument(s). " +
"Function requires at least 0 argument(s) " +
"and no more than 0 argument(s).");
}
public void testAbstractMethodHandling5() throws Exception {
testTypes(
"/** @type {!Function} */ var abstractFn = function() {};" +
"/** @param {number} x */ var f = abstractFn;" +
"f('x');",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testAbstractMethodHandling6() throws Exception {
testTypes(
"var goog = {};" +
"/** @type {Function} */ goog.abstractFn = function() {};" +
"/** @param {number} x */ goog.f = abstractFn;" +
"goog.f('x');",
"actual parameter 1 of goog.f does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testMethodInference1() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** @return {number} */ F.prototype.foo = function() { return 3; };" +
"/** @constructor \n * @extends {F} */ " +
"function G() {}" +
"/** @override */ G.prototype.foo = function() { return true; };",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testMethodInference2() throws Exception {
testTypes(
"var goog = {};" +
"/** @constructor */ goog.F = function() {};" +
"/** @return {number} */ goog.F.prototype.foo = " +
" function() { return 3; };" +
"/** @constructor \n * @extends {goog.F} */ " +
"goog.G = function() {};" +
"/** @override */ goog.G.prototype.foo = function() { return true; };",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testMethodInference3() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** @param {boolean} x \n * @return {number} */ " +
"F.prototype.foo = function(x) { return 3; };" +
"/** @constructor \n * @extends {F} */ " +
"function G() {}" +
"/** @override */ " +
"G.prototype.foo = function(x) { return x; };",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testMethodInference4() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** @param {boolean} x \n * @return {number} */ " +
"F.prototype.foo = function(x) { return 3; };" +
"/** @constructor \n * @extends {F} */ " +
"function G() {}" +
"/** @override */ " +
"G.prototype.foo = function(y) { return y; };",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testMethodInference5() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** @param {number} x \n * @return {string} */ " +
"F.prototype.foo = function(x) { return 'x'; };" +
"/** @constructor \n * @extends {F} */ " +
"function G() {}" +
"/** @type {number} */ G.prototype.num = 3;" +
"/** @override */ " +
"G.prototype.foo = function(y) { return this.num + y; };",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testMethodInference6() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** @param {number} x */ F.prototype.foo = function(x) { };" +
"/** @constructor \n * @extends {F} */ " +
"function G() {}" +
"/** @override */ G.prototype.foo = function() { };" +
"(new G()).foo(1);");
}
public void testMethodInference7() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"F.prototype.foo = function() { };" +
"/** @constructor \n * @extends {F} */ " +
"function G() {}" +
"/** @override */ G.prototype.foo = function(x, y) { };",
"mismatch of the foo property type and the type of the property " +
"it overrides from superclass F\n" +
"original: function (this:F): undefined\n" +
"override: function (this:G, ?, ?): undefined");
}
public void testMethodInference8() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"F.prototype.foo = function() { };" +
"/** @constructor \n * @extends {F} */ " +
"function G() {}" +
"/** @override */ " +
"G.prototype.foo = function(opt_b, var_args) { };" +
"(new G()).foo(1, 2, 3);");
}
public void testMethodInference9() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"F.prototype.foo = function() { };" +
"/** @constructor \n * @extends {F} */ " +
"function G() {}" +
"/** @override */ " +
"G.prototype.foo = function(var_args, opt_b) { };",
"variable length argument must be last");
}
public void testStaticMethodDeclaration1() throws Exception {
testTypes(
"/** @constructor */ function F() { F.foo(true); }" +
"/** @param {number} x */ F.foo = function(x) {};",
"actual parameter 1 of F.foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testStaticMethodDeclaration2() throws Exception {
testTypes(
"var goog = goog || {}; function f() { goog.foo(true); }" +
"/** @param {number} x */ goog.foo = function(x) {};",
"actual parameter 1 of goog.foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testStaticMethodDeclaration3() throws Exception {
testTypes(
"var goog = goog || {}; function f() { goog.foo(true); }" +
"goog.foo = function() {};",
"Function goog.foo: called with 1 argument(s). Function requires " +
"at least 0 argument(s) and no more than 0 argument(s).");
}
public void testDuplicateStaticMethodDecl1() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @param {number} x */ goog.foo = function(x) {};" +
"/** @param {number} x */ goog.foo = function(x) {};",
"variable goog.foo redefined with type function (number): undefined, " +
"original definition at [testcode]:1 " +
"with type function (number): undefined");
}
public void testDuplicateStaticMethodDecl2() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @param {number} x */ goog.foo = function(x) {};" +
"/** @param {number} x \n * @suppress {duplicate} */ " +
"goog.foo = function(x) {};");
}
public void testDuplicateStaticMethodDecl3() throws Exception {
testTypes(
"var goog = goog || {};" +
"goog.foo = function(x) {};" +
"goog.foo = function(x) {};");
}
public void testDuplicateStaticMethodDecl4() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @type {Function} */ goog.foo = function(x) {};" +
"goog.foo = function(x) {};");
}
public void testDuplicateStaticMethodDecl5() throws Exception {
testTypes(
"var goog = goog || {};" +
"goog.foo = function(x) {};" +
"/** @return {undefined} */ goog.foo = function(x) {};",
"variable goog.foo redefined with type function (?): undefined, " +
"original definition at [testcode]:1 with type " +
"function (?): undefined");
}
public void testDuplicateStaticPropertyDecl1() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @type {Foo} */ goog.foo;" +
"/** @type {Foo} */ goog.foo;" +
"/** @constructor */ function Foo() {}");
}
public void testDuplicateStaticPropertyDecl2() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @type {Foo} */ goog.foo;" +
"/** @type {Foo} \n * @suppress {duplicate} */ goog.foo;" +
"/** @constructor */ function Foo() {}");
}
public void testDuplicateStaticPropertyDecl3() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @type {!Foo} */ goog.foo;" +
"/** @type {string} */ goog.foo;" +
"/** @constructor */ function Foo() {}",
"variable goog.foo redefined with type string, " +
"original definition at [testcode]:1 with type Foo");
}
public void testDuplicateStaticPropertyDecl4() throws Exception {
testClosureTypesMultipleWarnings(
"var goog = goog || {};" +
"/** @type {!Foo} */ goog.foo;" +
"/** @type {string} */ goog.foo = 'x';" +
"/** @constructor */ function Foo() {}",
Lists.newArrayList(
"assignment to property foo of goog\n" +
"found : string\n" +
"required: Foo",
"variable goog.foo redefined with type string, " +
"original definition at [testcode]:1 with type Foo"));
}
public void testDuplicateStaticPropertyDecl5() throws Exception {
testClosureTypesMultipleWarnings(
"var goog = goog || {};" +
"/** @type {!Foo} */ goog.foo;" +
"/** @type {string}\n * @suppress {duplicate} */ goog.foo = 'x';" +
"/** @constructor */ function Foo() {}",
Lists.newArrayList(
"assignment to property foo of goog\n" +
"found : string\n" +
"required: Foo",
"variable goog.foo redefined with type string, " +
"original definition at [testcode]:1 with type Foo"));
}
public void testDuplicateStaticPropertyDecl6() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @type {string} */ goog.foo = 'y';" +
"/** @type {string}\n * @suppress {duplicate} */ goog.foo = 'x';");
}
public void testDuplicateStaticPropertyDecl7() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @param {string} x */ goog.foo;" +
"/** @type {function(string)} */ goog.foo;");
}
public void testDuplicateStaticPropertyDecl8() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @return {EventCopy} */ goog.foo;" +
"/** @constructor */ function EventCopy() {}" +
"/** @return {EventCopy} */ goog.foo;");
}
public void testDuplicateStaticPropertyDecl9() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @return {EventCopy} */ goog.foo;" +
"/** @return {EventCopy} */ goog.foo;" +
"/** @constructor */ function EventCopy() {}");
}
public void testDuplicateStaticPropertyDec20() throws Exception {
testTypes(
"/**\n" +
" * @fileoverview\n" +
" * @suppress {duplicate}\n" +
" */" +
"var goog = goog || {};" +
"/** @type {string} */ goog.foo = 'y';" +
"/** @type {string} */ goog.foo = 'x';");
}
public void testDuplicateLocalVarDecl() throws Exception {
testClosureTypesMultipleWarnings(
"/** @param {number} x */\n" +
"function f(x) { /** @type {string} */ var x = ''; }",
Lists.newArrayList(
"variable x redefined with type string, original definition" +
" at [testcode]:2 with type number",
"initializing variable\n" +
"found : string\n" +
"required: number"));
}
public void testDuplicateInstanceMethod1() throws Exception {
// If there's no jsdoc on the methods, then we treat them like
// any other inferred properties.
testTypes(
"/** @constructor */ function F() {}" +
"F.prototype.bar = function() {};" +
"F.prototype.bar = function() {};");
}
public void testDuplicateInstanceMethod2() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** jsdoc */ F.prototype.bar = function() {};" +
"/** jsdoc */ F.prototype.bar = function() {};",
"variable F.prototype.bar redefined with type " +
"function (this:F): undefined, original definition at " +
"[testcode]:1 with type function (this:F): undefined");
}
public void testDuplicateInstanceMethod3() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"F.prototype.bar = function() {};" +
"/** jsdoc */ F.prototype.bar = function() {};",
"variable F.prototype.bar redefined with type " +
"function (this:F): undefined, original definition at " +
"[testcode]:1 with type function (this:F): undefined");
}
public void testDuplicateInstanceMethod4() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** jsdoc */ F.prototype.bar = function() {};" +
"F.prototype.bar = function() {};");
}
public void testDuplicateInstanceMethod5() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** jsdoc \n * @return {number} */ F.prototype.bar = function() {" +
" return 3;" +
"};" +
"/** jsdoc \n * @suppress {duplicate} */ " +
"F.prototype.bar = function() { return ''; };",
"inconsistent return type\n" +
"found : string\n" +
"required: number");
}
public void testDuplicateInstanceMethod6() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** jsdoc \n * @return {number} */ F.prototype.bar = function() {" +
" return 3;" +
"};" +
"/** jsdoc \n * @return {string} * \n @suppress {duplicate} */ " +
"F.prototype.bar = function() { return ''; };",
"assignment to property bar of F.prototype\n" +
"found : function (this:F): string\n" +
"required: function (this:F): number");
}
public void testStubFunctionDeclaration1() throws Exception {
testFunctionType(
"/** @constructor */ function f() {};" +
"/** @param {number} x \n * @param {string} y \n" +
" * @return {number} */ f.prototype.foo;",
"(new f).foo",
"function (this:f, number, string): number");
}
public void testStubFunctionDeclaration2() throws Exception {
testExternFunctionType(
// externs
"/** @constructor */ function f() {};" +
"/** @constructor \n * @extends {f} */ f.subclass;",
"f.subclass",
"function (new:f.subclass): ?");
}
public void testStubFunctionDeclaration3() throws Exception {
testFunctionType(
"/** @constructor */ function f() {};" +
"/** @return {undefined} */ f.foo;",
"f.foo",
"function (): undefined");
}
public void testStubFunctionDeclaration4() throws Exception {
testFunctionType(
"/** @constructor */ function f() { " +
" /** @return {number} */ this.foo;" +
"}",
"(new f).foo",
"function (this:f): number");
}
public void testStubFunctionDeclaration5() throws Exception {
testFunctionType(
"/** @constructor */ function f() { " +
" /** @type {Function} */ this.foo;" +
"}",
"(new f).foo",
createNullableType(U2U_CONSTRUCTOR_TYPE).toString());
}
public void testStubFunctionDeclaration6() throws Exception {
testFunctionType(
"/** @constructor */ function f() {} " +
"/** @type {Function} */ f.prototype.foo;",
"(new f).foo",
createNullableType(U2U_CONSTRUCTOR_TYPE).toString());
}
public void testStubFunctionDeclaration7() throws Exception {
testFunctionType(
"/** @constructor */ function f() {} " +
"/** @type {Function} */ f.prototype.foo = function() {};",
"(new f).foo",
createNullableType(U2U_CONSTRUCTOR_TYPE).toString());
}
public void testStubFunctionDeclaration8() throws Exception {
testFunctionType(
"/** @type {Function} */ var f = function() {}; ",
"f",
createNullableType(U2U_CONSTRUCTOR_TYPE).toString());
}
public void testStubFunctionDeclaration9() throws Exception {
testFunctionType(
"/** @type {function():number} */ var f; ",
"f",
"function (): number");
}
public void testStubFunctionDeclaration10() throws Exception {
testFunctionType(
"/** @type {function(number):number} */ var f = function(x) {};",
"f",
"function (number): number");
}
public void testNestedFunctionInference1() throws Exception {
String nestedAssignOfFooAndBar =
"/** @constructor */ function f() {};" +
"f.prototype.foo = f.prototype.bar = function(){};";
testFunctionType(nestedAssignOfFooAndBar, "(new f).bar",
"function (this:f): undefined");
}
/**
* Tests the type of a function definition. The function defined by
* {@code functionDef} should be named {@code "f"}.
*/
private void testFunctionType(String functionDef, String functionType)
throws Exception {
testFunctionType(functionDef, "f", functionType);
}
/**
* Tests the type of a function definition. The function defined by
* {@code functionDef} should be named {@code functionName}.
*/
private void testFunctionType(String functionDef, String functionName,
String functionType) throws Exception {
// using the variable initialization check to verify the function's type
testTypes(
functionDef +
"/** @type number */var a=" + functionName + ";",
"initializing variable\n" +
"found : " + functionType + "\n" +
"required: number");
}
/**
* Tests the type of a function definition in externs.
* The function defined by {@code functionDef} should be
* named {@code functionName}.
*/
private void testExternFunctionType(String functionDef, String functionName,
String functionType) throws Exception {
testTypes(
functionDef,
"/** @type number */var a=" + functionName + ";",
"initializing variable\n" +
"found : " + functionType + "\n" +
"required: number", false);
}
public void testTypeRedefinition() throws Exception {
testTypes("a={};/**@enum {string}*/ a.A = {ZOR:'b'};"
+ "/** @constructor */ a.A = function() {}",
"variable a.A redefined with type function (new:a.A): undefined, " +
"original definition at [testcode]:1 with type enum{a.A}");
}
public void testIn1() throws Exception {
testTypes("'foo' in Object");
}
public void testIn2() throws Exception {
testTypes("3 in Object");
}
public void testIn3() throws Exception {
testTypes("undefined in Object");
}
public void testIn4() throws Exception {
testTypes("Date in Object",
"left side of 'in'\n" +
"found : function (new:Date, ?=, ?=, ?=, ?=, ?=, ?=, ?=): string\n" +
"required: string");
}
public void testIn5() throws Exception {
testTypes("'x' in null",
"'in' requires an object\n" +
"found : null\n" +
"required: Object");
}
public void testIn6() throws Exception {
testTypes(
"/** @param {number} x */" +
"function g(x) {}" +
"g(1 in {});",
"actual parameter 1 of g does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testIn7() throws Exception {
// Make sure we do inference in the 'in' expression.
testTypes(
"/**\n" +
" * @param {number} x\n" +
" * @return {number}\n" +
" */\n" +
"function g(x) { return 5; }" +
"function f() {" +
" var x = {};" +
" x.foo = '3';" +
" return g(x.foo) in {};" +
"}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testForIn1() throws Exception {
testTypes(
"/** @param {boolean} x */ function f(x) {}" +
"for (var k in {}) {" +
" f(k);" +
"}",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: boolean");
}
public void testForIn2() throws Exception {
testTypes(
"/** @param {boolean} x */ function f(x) {}" +
"/** @enum {string} */ var E = {FOO: 'bar'};" +
"/** @type {Object.<E, string>} */ var obj = {};" +
"var k = null;" +
"for (k in obj) {" +
" f(k);" +
"}",
"actual parameter 1 of f does not match formal parameter\n" +
"found : E.<string>\n" +
"required: boolean");
}
public void testForIn3() throws Exception {
testTypes(
"/** @param {boolean} x */ function f(x) {}" +
"/** @type {Object.<number>} */ var obj = {};" +
"for (var k in obj) {" +
" f(obj[k]);" +
"}",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: boolean");
}
public void testForIn4() throws Exception {
testTypes(
"/** @param {boolean} x */ function f(x) {}" +
"/** @enum {string} */ var E = {FOO: 'bar'};" +
"/** @type {Object.<E, Array>} */ var obj = {};" +
"for (var k in obj) {" +
" f(obj[k]);" +
"}",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (Array|null)\n" +
"required: boolean");
}
public void testForIn5() throws Exception {
testTypes(
"/** @param {boolean} x */ function f(x) {}" +
"/** @constructor */ var E = function(){};" +
"/** @type {Object.<E, number>} */ var obj = {};" +
"for (var k in obj) {" +
" f(k);" +
"}",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: boolean");
}
// TODO(nicksantos): change this to something that makes sense.
// public void testComparison1() throws Exception {
// testTypes("/**@type null */var a;" +
// "/**@type !Date */var b;" +
// "if (a==b) {}",
// "condition always evaluates to false\n" +
// "left : null\n" +
// "right: Date");
// }
public void testComparison2() throws Exception {
testTypes("/**@type number*/var a;" +
"/**@type !Date */var b;" +
"if (a!==b) {}",
"condition always evaluates to the same value\n" +
"left : number\n" +
"right: Date");
}
public void testComparison3() throws Exception {
// Since null == undefined in JavaScript, this code is reasonable.
testTypes("/** @type {(Object,undefined)} */var a;" +
"var b = a == null");
}
public void testComparison4() throws Exception {
testTypes("/** @type {(!Object,undefined)} */var a;" +
"/** @type {!Object} */var b;" +
"var c = a == b");
}
public void testComparison5() throws Exception {
testTypes("/** @type null */var a;" +
"/** @type null */var b;" +
"a == b",
"condition always evaluates to true\n" +
"left : null\n" +
"right: null");
}
public void testComparison6() throws Exception {
testTypes("/** @type null */var a;" +
"/** @type null */var b;" +
"a != b",
"condition always evaluates to false\n" +
"left : null\n" +
"right: null");
}
public void testComparison7() throws Exception {
testTypes("var a;" +
"var b;" +
"a == b",
"condition always evaluates to true\n" +
"left : undefined\n" +
"right: undefined");
}
public void testComparison8() throws Exception {
testTypes("/** @type {Array.<string>} */ var a = [];" +
"a[0] == null || a[1] == undefined");
}
public void testComparison9() throws Exception {
testTypes("/** @type {Array.<undefined>} */ var a = [];" +
"a[0] == null",
"condition always evaluates to true\n" +
"left : undefined\n" +
"right: null");
}
public void testComparison10() throws Exception {
testTypes("/** @type {Array.<undefined>} */ var a = [];" +
"a[0] === null");
}
public void testComparison11() throws Exception {
testTypes(
"(function(){}) == 'x'",
"condition always evaluates to false\n" +
"left : function (): undefined\n" +
"right: string");
}
public void testComparison12() throws Exception {
testTypes(
"(function(){}) == 3",
"condition always evaluates to false\n" +
"left : function (): undefined\n" +
"right: number");
}
public void testComparison13() throws Exception {
testTypes(
"(function(){}) == false",
"condition always evaluates to false\n" +
"left : function (): undefined\n" +
"right: boolean");
}
public void testDeleteOperator1() throws Exception {
testTypes(
"var x = {};" +
"/** @return {string} */ function f() { return delete x['a']; }",
"inconsistent return type\n" +
"found : boolean\n" +
"required: string");
}
public void testDeleteOperator2() throws Exception {
testTypes(
"var obj = {};" +
"/** \n" +
" * @param {string} x\n" +
" * @return {Object} */ function f(x) { return obj; }" +
"/** @param {?number} x */ function g(x) {" +
" if (x) { delete f(x)['a']; }" +
"}",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testEnumStaticMethod1() throws Exception {
testTypes(
"/** @enum */ var Foo = {AAA: 1};" +
"/** @param {number} x */ Foo.method = function(x) {};" +
"Foo.method(true);",
"actual parameter 1 of Foo.method does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testEnumStaticMethod2() throws Exception {
testTypes(
"/** @enum */ var Foo = {AAA: 1};" +
"/** @param {number} x */ Foo.method = function(x) {};" +
"function f() { Foo.method(true); }",
"actual parameter 1 of Foo.method does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testEnum1() throws Exception {
testTypes("/**@enum*/var a={BB:1,CC:2};\n" +
"/**@type {a}*/var d;d=a.BB;");
}
public void testEnum2() throws Exception {
testTypes("/**@enum*/var a={b:1}",
"enum key b must be a syntactic constant");
}
public void testEnum3() throws Exception {
testTypes("/**@enum*/var a={BB:1,BB:2}",
"variable a.BB redefined with type a.<number>, " +
"original definition at [testcode]:1 with type a.<number>");
}
public void testEnum4() throws Exception {
testTypes("/**@enum*/var a={BB:'string'}",
"assignment to property BB of enum{a}\n" +
"found : string\n" +
"required: number");
}
public void testEnum5() throws Exception {
testTypes("/**@enum {String}*/var a={BB:'string'}",
"assignment to property BB of enum{a}\n" +
"found : string\n" +
"required: (String|null)");
}
public void testEnum6() throws Exception {
testTypes("/**@enum*/var a={BB:1,CC:2};\n/**@type {!Array}*/var d;d=a.BB;",
"assignment\n" +
"found : a.<number>\n" +
"required: Array");
}
public void testEnum7() throws Exception {
testTypes("/** @enum */var a={AA:1,BB:2,CC:3};" +
"/** @type a */var b=a.D;",
"element D does not exist on this enum");
}
public void testEnum8() throws Exception {
testClosureTypesMultipleWarnings("/** @enum */var a=8;",
Lists.newArrayList(
"enum initializer must be an object literal or an enum",
"initializing variable\n" +
"found : number\n" +
"required: enum{a}"));
}
public void testEnum9() throws Exception {
testClosureTypesMultipleWarnings(
"var goog = {};" +
"/** @enum */goog.a=8;",
Lists.newArrayList(
"assignment to property a of goog\n" +
"found : number\n" +
"required: enum{goog.a}",
"enum initializer must be an object literal or an enum"));
}
public void testEnum10() throws Exception {
testTypes(
"/** @enum {number} */" +
"goog.K = { A : 3 };");
}
public void testEnum11() throws Exception {
testTypes(
"/** @enum {number} */" +
"goog.K = { 502 : 3 };");
}
public void testEnum12() throws Exception {
testTypes(
"/** @enum {number} */ var a = {};" +
"/** @enum */ var b = a;");
}
public void testEnum13() throws Exception {
testTypes(
"/** @enum {number} */ var a = {};" +
"/** @enum {string} */ var b = a;",
"incompatible enum element types\n" +
"found : number\n" +
"required: string");
}
public void testEnum14() throws Exception {
testTypes(
"/** @enum {number} */ var a = {FOO:5};" +
"/** @enum */ var b = a;" +
"var c = b.FOO;");
}
public void testEnum15() throws Exception {
testTypes(
"/** @enum {number} */ var a = {FOO:5};" +
"/** @enum */ var b = a;" +
"var c = b.BAR;",
"element BAR does not exist on this enum");
}
public void testEnum16() throws Exception {
testTypes("var goog = {};" +
"/**@enum*/goog .a={BB:1,BB:2}",
"variable goog.a.BB redefined with type goog.a.<number>, " +
"original definition at [testcode]:1 with type goog.a.<number>");
}
public void testEnum17() throws Exception {
testTypes("var goog = {};" +
"/**@enum*/goog.a={BB:'string'}",
"assignment to property BB of enum{goog.a}\n" +
"found : string\n" +
"required: number");
}
public void testEnum18() throws Exception {
testTypes("/**@enum*/ var E = {A: 1, B: 2};" +
"/** @param {!E} x\n@return {number} */\n" +
"var f = function(x) { return x; };");
}
public void testEnum19() throws Exception {
testTypes("/**@enum*/ var E = {A: 1, B: 2};" +
"/** @param {number} x\n@return {!E} */\n" +
"var f = function(x) { return x; };",
"inconsistent return type\n" +
"found : number\n" +
"required: E.<number>");
}
public void testEnum20() throws Exception {
testTypes("/**@enum*/ var E = {A: 1, B: 2}; var x = []; x[E.A] = 0;");
}
public void testEnum21() throws Exception {
Node n = parseAndTypeCheck(
"/** @enum {string} */ var E = {A : 'a', B : 'b'};\n" +
"/** @param {!E} x\n@return {!E} */ function f(x) { return x; }");
Node nodeX = n.getLastChild().getLastChild().getLastChild().getLastChild();
JSType typeE = nodeX.getJSType();
assertFalse(typeE.isObject());
assertFalse(typeE.isNullable());
}
public void testEnum22() throws Exception {
testTypes("/**@enum*/ var E = {A: 1, B: 2};" +
"/** @param {E} x \n* @return {number} */ function f(x) {return x}");
}
public void testEnum23() throws Exception {
testTypes("/**@enum*/ var E = {A: 1, B: 2};" +
"/** @param {E} x \n* @return {string} */ function f(x) {return x}",
"inconsistent return type\n" +
"found : E.<number>\n" +
"required: string");
}
public void testEnum24() throws Exception {
testTypes("/**@enum {Object} */ var E = {A: {}};" +
"/** @param {E} x \n* @return {!Object} */ function f(x) {return x}",
"inconsistent return type\n" +
"found : E.<(Object|null)>\n" +
"required: Object");
}
public void testEnum25() throws Exception {
testTypes("/**@enum {!Object} */ var E = {A: {}};" +
"/** @param {E} x \n* @return {!Object} */ function f(x) {return x}");
}
public void testEnum26() throws Exception {
testTypes("var a = {}; /**@enum*/ a.B = {A: 1, B: 2};" +
"/** @param {a.B} x \n* @return {number} */ function f(x) {return x}");
}
public void testEnum27() throws Exception {
// x is unknown
testTypes("/** @enum */ var A = {B: 1, C: 2}; " +
"function f(x) { return A == x; }");
}
public void testEnum28() throws Exception {
// x is unknown
testTypes("/** @enum */ var A = {B: 1, C: 2}; " +
"function f(x) { return A.B == x; }");
}
public void testEnum29() throws Exception {
testTypes("/** @enum */ var A = {B: 1, C: 2}; " +
"/** @return {number} */ function f() { return A; }",
"inconsistent return type\n" +
"found : enum{A}\n" +
"required: number");
}
public void testEnum30() throws Exception {
testTypes("/** @enum */ var A = {B: 1, C: 2}; " +
"/** @return {number} */ function f() { return A.B; }");
}
public void testEnum31() throws Exception {
testTypes("/** @enum */ var A = {B: 1, C: 2}; " +
"/** @return {A} */ function f() { return A; }",
"inconsistent return type\n" +
"found : enum{A}\n" +
"required: A.<number>");
}
public void testEnum32() throws Exception {
testTypes("/** @enum */ var A = {B: 1, C: 2}; " +
"/** @return {A} */ function f() { return A.B; }");
}
public void testEnum34() throws Exception {
testTypes("/** @enum */ var A = {B: 1, C: 2}; " +
"/** @param {number} x */ function f(x) { return x == A.B; }");
}
public void testEnum35() throws Exception {
testTypes("var a = a || {}; /** @enum */ a.b = {C: 1, D: 2};" +
"/** @return {a.b} */ function f() { return a.b.C; }");
}
public void testEnum36() throws Exception {
testTypes("var a = a || {}; /** @enum */ a.b = {C: 1, D: 2};" +
"/** @return {!a.b} */ function f() { return 1; }",
"inconsistent return type\n" +
"found : number\n" +
"required: a.b.<number>");
}
public void testEnum37() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @enum {number} */ goog.a = {};" +
"/** @enum */ var b = goog.a;");
}
public void testEnum38() throws Exception {
testTypes(
"/** @enum {MyEnum} */ var MyEnum = {};" +
"/** @param {MyEnum} x */ function f(x) {}",
"Parse error. Cycle detected in inheritance chain " +
"of type MyEnum");
}
public void testEnum39() throws Exception {
testTypes(
"/** @enum {Number} */ var MyEnum = {FOO: new Number(1)};" +
"/** @param {MyEnum} x \n * @return {number} */" +
"function f(x) { return x == MyEnum.FOO && MyEnum.FOO == x; }",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testEnum40() throws Exception {
testTypes(
"/** @enum {Number} */ var MyEnum = {FOO: new Number(1)};" +
"/** @param {number} x \n * @return {number} */" +
"function f(x) { return x == MyEnum.FOO && MyEnum.FOO == x; }",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testEnum41() throws Exception {
testTypes(
"/** @enum {number} */ var MyEnum = {/** @const */ FOO: 1};" +
"/** @return {string} */" +
"function f() { return MyEnum.FOO; }",
"inconsistent return type\n" +
"found : MyEnum.<number>\n" +
"required: string");
}
public void testEnum42() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"/** @enum {Object} */ var MyEnum = {FOO: {newProperty: 1, b: 2}};" +
"f(MyEnum.FOO.newProperty);");
}
public void testAliasedEnum1() throws Exception {
testTypes(
"/** @enum */ var YourEnum = {FOO: 3};" +
"/** @enum */ var MyEnum = YourEnum;" +
"/** @param {MyEnum} x */ function f(x) {} f(MyEnum.FOO);");
}
public void testAliasedEnum2() throws Exception {
testTypes(
"/** @enum */ var YourEnum = {FOO: 3};" +
"/** @enum */ var MyEnum = YourEnum;" +
"/** @param {YourEnum} x */ function f(x) {} f(MyEnum.FOO);");
}
public void testAliasedEnum3() throws Exception {
testTypes(
"/** @enum */ var YourEnum = {FOO: 3};" +
"/** @enum */ var MyEnum = YourEnum;" +
"/** @param {MyEnum} x */ function f(x) {} f(YourEnum.FOO);");
}
public void testAliasedEnum4() throws Exception {
testTypes(
"/** @enum */ var YourEnum = {FOO: 3};" +
"/** @enum */ var MyEnum = YourEnum;" +
"/** @param {YourEnum} x */ function f(x) {} f(YourEnum.FOO);");
}
public void testAliasedEnum5() throws Exception {
testTypes(
"/** @enum */ var YourEnum = {FOO: 3};" +
"/** @enum */ var MyEnum = YourEnum;" +
"/** @param {string} x */ function f(x) {} f(MyEnum.FOO);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : YourEnum.<number>\n" +
"required: string");
}
public void testBackwardsEnumUse1() throws Exception {
testTypes(
"/** @return {string} */ function f() { return MyEnum.FOO; }" +
"/** @enum {string} */ var MyEnum = {FOO: 'x'};");
}
public void testBackwardsEnumUse2() throws Exception {
testTypes(
"/** @return {number} */ function f() { return MyEnum.FOO; }" +
"/** @enum {string} */ var MyEnum = {FOO: 'x'};",
"inconsistent return type\n" +
"found : MyEnum.<string>\n" +
"required: number");
}
public void testBackwardsEnumUse3() throws Exception {
testTypes(
"/** @return {string} */ function f() { return MyEnum.FOO; }" +
"/** @enum {string} */ var YourEnum = {FOO: 'x'};" +
"/** @enum {string} */ var MyEnum = YourEnum;");
}
public void testBackwardsEnumUse4() throws Exception {
testTypes(
"/** @return {number} */ function f() { return MyEnum.FOO; }" +
"/** @enum {string} */ var YourEnum = {FOO: 'x'};" +
"/** @enum {string} */ var MyEnum = YourEnum;",
"inconsistent return type\n" +
"found : YourEnum.<string>\n" +
"required: number");
}
public void testBackwardsEnumUse5() throws Exception {
testTypes(
"/** @return {string} */ function f() { return MyEnum.BAR; }" +
"/** @enum {string} */ var YourEnum = {FOO: 'x'};" +
"/** @enum {string} */ var MyEnum = YourEnum;",
"element BAR does not exist on this enum");
}
public void testBackwardsTypedefUse1() throws Exception {
testTypes(
"/** @this {MyTypedef} */ function f() {}" +
"/** @typedef {string} */ var MyTypedef;",
"@this type of a function must be an object\n" +
"Actual type: string");
}
public void testBackwardsTypedefUse2() throws Exception {
testTypes(
"/** @this {MyTypedef} */ function f() {}" +
"/** @typedef {!(Date|Array)} */ var MyTypedef;");
}
public void testBackwardsTypedefUse3() throws Exception {
testTypes(
"/** @this {MyTypedef} */ function f() {}" +
"/** @typedef {(Date|string)} */ var MyTypedef;",
"@this type of a function must be an object\n" +
"Actual type: (Date|null|string)");
}
public void testBackwardsTypedefUse4() throws Exception {
testTypes(
"/** @return {MyTypedef} */ function f() { return null; }" +
"/** @typedef {string} */ var MyTypedef;",
"inconsistent return type\n" +
"found : null\n" +
"required: string");
}
public void testBackwardsTypedefUse6() throws Exception {
testTypes(
"/** @return {goog.MyTypedef} */ function f() { return null; }" +
"var goog = {};" +
"/** @typedef {string} */ goog.MyTypedef;",
"inconsistent return type\n" +
"found : null\n" +
"required: string");
}
public void testBackwardsTypedefUse7() throws Exception {
testTypes(
"/** @return {goog.MyTypedef} */ function f() { return null; }" +
"var goog = {};" +
"/** @typedef {Object} */ goog.MyTypedef;");
}
public void testBackwardsTypedefUse8() throws Exception {
// Tehnically, this isn't quite right, because the JS runtime
// will coerce null -> the global object. But we'll punt on that for now.
testTypes(
"/** @param {!Array} x */ function g(x) {}" +
"/** @this {goog.MyTypedef} */ function f() { g(this); }" +
"var goog = {};" +
"/** @typedef {(Array|null|undefined)} */ goog.MyTypedef;");
}
public void testBackwardsTypedefUse9() throws Exception {
testTypes(
"/** @param {!Array} x */ function g(x) {}" +
"/** @this {goog.MyTypedef} */ function f() { g(this); }" +
"var goog = {};" +
"/** @typedef {(Error|null|undefined)} */ goog.MyTypedef;",
"actual parameter 1 of g does not match formal parameter\n" +
"found : Error\n" +
"required: Array");
}
public void testBackwardsTypedefUse10() throws Exception {
testTypes(
"/** @param {goog.MyEnum} x */ function g(x) {}" +
"var goog = {};" +
"/** @enum {goog.MyTypedef} */ goog.MyEnum = {FOO: 1};" +
"/** @typedef {number} */ goog.MyTypedef;" +
"g(1);",
"actual parameter 1 of g does not match formal parameter\n" +
"found : number\n" +
"required: goog.MyEnum.<number>");
}
public void testBackwardsConstructor1() throws Exception {
testTypes(
"function f() { (new Foo(true)); }" +
"/** \n * @constructor \n * @param {number} x */" +
"var Foo = function(x) {};",
"actual parameter 1 of Foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testBackwardsConstructor2() throws Exception {
testTypes(
"function f() { (new Foo(true)); }" +
"/** \n * @constructor \n * @param {number} x */" +
"var YourFoo = function(x) {};" +
"/** \n * @constructor \n * @param {number} x */" +
"var Foo = YourFoo;",
"actual parameter 1 of Foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testMinimalConstructorAnnotation() throws Exception {
testTypes("/** @constructor */function Foo(){}");
}
public void testGoodExtends1() throws Exception {
// A minimal @extends example
testTypes("/** @constructor */function base() {}\n" +
"/** @constructor\n * @extends {base} */function derived() {}\n");
}
public void testGoodExtends2() throws Exception {
testTypes("/** @constructor\n * @extends base */function derived() {}\n" +
"/** @constructor */function base() {}\n");
}
public void testGoodExtends3() throws Exception {
testTypes("/** @constructor\n * @extends {Object} */function base() {}\n" +
"/** @constructor\n * @extends {base} */function derived() {}\n");
}
public void testGoodExtends4() throws Exception {
// Ensure that @extends actually sets the base type of a constructor
// correctly. Because this isn't part of the human-readable Function
// definition, we need to crawl the prototype chain (eww).
Node n = parseAndTypeCheck(
"var goog = {};\n" +
"/** @constructor */goog.Base = function(){};\n" +
"/** @constructor\n" +
" * @extends {goog.Base} */goog.Derived = function(){};\n");
Node subTypeName = n.getLastChild().getLastChild().getFirstChild();
assertEquals("goog.Derived", subTypeName.getQualifiedName());
FunctionType subCtorType =
(FunctionType) subTypeName.getNext().getJSType();
assertEquals("goog.Derived", subCtorType.getInstanceType().toString());
JSType superType = subCtorType.getPrototype().getImplicitPrototype();
assertEquals("goog.Base", superType.toString());
}
public void testGoodExtends5() throws Exception {
// we allow for the extends annotation to be placed first
testTypes("/** @constructor */function base() {}\n" +
"/** @extends {base}\n * @constructor */function derived() {}\n");
}
public void testGoodExtends6() throws Exception {
testFunctionType(
CLOSURE_DEFS +
"/** @constructor */function base() {}\n" +
"/** @return {number} */ " +
" base.prototype.foo = function() { return 1; };\n" +
"/** @extends {base}\n * @constructor */function derived() {}\n" +
"goog.inherits(derived, base);",
"derived.superClass_.foo",
"function (this:base): number");
}
public void testGoodExtends7() throws Exception {
testFunctionType(
"Function.prototype.inherits = function(x) {};" +
"/** @constructor */function base() {}\n" +
"/** @extends {base}\n * @constructor */function derived() {}\n" +
"derived.inherits(base);",
"(new derived).constructor",
"function (new:derived): undefined");
}
public void testGoodExtends8() throws Exception {
testTypes("/** @constructor \n @extends {Base} */ function Sub() {}" +
"/** @return {number} */ function f() { return (new Sub()).foo; }" +
"/** @constructor */ function Base() {}" +
"/** @type {boolean} */ Base.prototype.foo = true;",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testGoodExtends9() throws Exception {
testTypes(
"/** @constructor */ function Super() {}" +
"Super.prototype.foo = function() {};" +
"/** @constructor \n * @extends {Super} */ function Sub() {}" +
"Sub.prototype = new Super();" +
"/** @override */ Sub.prototype.foo = function() {};");
}
public void testGoodExtends10() throws Exception {
testTypes(
"/** @constructor */ function Super() {}" +
"/** @constructor \n * @extends {Super} */ function Sub() {}" +
"Sub.prototype = new Super();" +
"/** @return {Super} */ function foo() { return new Sub(); }");
}
public void testGoodExtends11() throws Exception {
testTypes(
"/** @constructor */ function Super() {}" +
"/** @param {boolean} x */ Super.prototype.foo = function(x) {};" +
"/** @constructor \n * @extends {Super} */ function Sub() {}" +
"Sub.prototype = new Super();" +
"(new Sub()).foo(0);",
"actual parameter 1 of Super.prototype.foo " +
"does not match formal parameter\n" +
"found : number\n" +
"required: boolean");
}
public void testGoodExtends12() throws Exception {
testTypes(
"/** @constructor \n * @extends {Super} */ function Sub() {}" +
"/** @constructor \n * @extends {Sub} */ function Sub2() {}" +
"/** @constructor */ function Super() {}" +
"/** @param {Super} x */ function foo(x) {}" +
"foo(new Sub2());");
}
public void testGoodExtends13() throws Exception {
testTypes(
"/** @constructor \n * @extends {B} */ function C() {}" +
"/** @constructor \n * @extends {D} */ function E() {}" +
"/** @constructor \n * @extends {C} */ function D() {}" +
"/** @constructor \n * @extends {A} */ function B() {}" +
"/** @constructor */ function A() {}" +
"/** @param {number} x */ function f(x) {} f(new E());",
"actual parameter 1 of f does not match formal parameter\n" +
"found : E\n" +
"required: number");
}
public void testGoodExtends14() throws Exception {
testTypes(
CLOSURE_DEFS +
"/** @param {Function} f */ function g(f) {" +
" /** @constructor */ function NewType() {};" +
" goog.inherits(NewType, f);" +
" (new NewType());" +
"}");
}
public void testGoodExtends15() throws Exception {
testTypes(
CLOSURE_DEFS +
"/** @constructor */ function OldType() {}" +
"/** @param {?function(new:OldType)} f */ function g(f) {" +
" /**\n" +
" * @constructor\n" +
" * @extends {OldType}\n" +
" */\n" +
" function NewType() {};" +
" goog.inherits(NewType, f);" +
" NewType.prototype.method = function() {" +
" NewType.superClass_.foo.call(this);" +
" };" +
"}",
"Property foo never defined on OldType.prototype");
}
public void testGoodExtends16() throws Exception {
testTypes(
CLOSURE_DEFS +
"/** @param {Function} f */ function g(f) {" +
" /** @constructor */ function NewType() {};" +
" goog.inherits(f, NewType);" +
" (new NewType());" +
"}");
}
public void testBadExtends1() throws Exception {
testTypes("/** @constructor */function base() {}\n" +
"/** @constructor\n * @extends {not_base} */function derived() {}\n",
"Bad type annotation. Unknown type not_base");
}
public void testBadExtends2() throws Exception {
testTypes("/** @constructor */function base() {\n" +
"/** @type {!Number}*/\n" +
"this.baseMember = new Number(4);\n" +
"}\n" +
"/** @constructor\n" +
" * @extends {base} */function derived() {}\n" +
"/** @param {!String} x*/\n" +
"function foo(x){ }\n" +
"/** @type {!derived}*/var y;\n" +
"foo(y.baseMember);\n",
"actual parameter 1 of foo does not match formal parameter\n" +
"found : Number\n" +
"required: String");
}
public void testBadExtends3() throws Exception {
testTypes("/** @extends {Object} */function base() {}",
"@extends used without @constructor or @interface for base");
}
public void testBadExtends4() throws Exception {
// If there's a subclass of a class with a bad extends,
// we only want to warn about the first one.
testTypes(
"/** @constructor \n * @extends {bad} */ function Sub() {}" +
"/** @constructor \n * @extends {Sub} */ function Sub2() {}" +
"/** @param {Sub} x */ function foo(x) {}" +
"foo(new Sub2());",
"Bad type annotation. Unknown type bad");
}
public void testLateExtends() throws Exception {
testTypes(
CLOSURE_DEFS +
"/** @constructor */ function Foo() {}\n" +
"Foo.prototype.foo = function() {};\n" +
"/** @constructor */function Bar() {}\n" +
"goog.inherits(Foo, Bar);\n",
"Missing @extends tag on type Foo");
}
public void testSuperclassMatch() throws Exception {
compiler.getOptions().setCodingConvention(new GoogleCodingConvention());
testTypes("/** @constructor */ var Foo = function() {};\n" +
"/** @constructor \n @extends Foo */ var Bar = function() {};\n" +
"Bar.inherits = function(x){};" +
"Bar.inherits(Foo);\n");
}
public void testSuperclassMatchWithMixin() throws Exception {
compiler.getOptions().setCodingConvention(new GoogleCodingConvention());
testTypes("/** @constructor */ var Foo = function() {};\n" +
"/** @constructor */ var Baz = function() {};\n" +
"/** @constructor \n @extends Foo */ var Bar = function() {};\n" +
"Bar.inherits = function(x){};" +
"Bar.mixin = function(y){};" +
"Bar.inherits(Foo);\n" +
"Bar.mixin(Baz);\n");
}
public void testSuperclassMismatch1() throws Exception {
compiler.getOptions().setCodingConvention(new GoogleCodingConvention());
testTypes("/** @constructor */ var Foo = function() {};\n" +
"/** @constructor \n @extends Object */ var Bar = function() {};\n" +
"Bar.inherits = function(x){};" +
"Bar.inherits(Foo);\n",
"Missing @extends tag on type Bar");
}
public void testSuperclassMismatch2() throws Exception {
compiler.getOptions().setCodingConvention(new GoogleCodingConvention());
testTypes("/** @constructor */ var Foo = function(){};\n" +
"/** @constructor */ var Bar = function(){};\n" +
"Bar.inherits = function(x){};" +
"Bar.inherits(Foo);",
"Missing @extends tag on type Bar");
}
public void testSuperClassDefinedAfterSubClass1() throws Exception {
testTypes(
"/** @constructor \n * @extends {Base} */ function A() {}" +
"/** @constructor \n * @extends {Base} */ function B() {}" +
"/** @constructor */ function Base() {}" +
"/** @param {A|B} x \n * @return {B|A} */ " +
"function foo(x) { return x; }");
}
public void testSuperClassDefinedAfterSubClass2() throws Exception {
testTypes(
"/** @constructor \n * @extends {Base} */ function A() {}" +
"/** @constructor \n * @extends {Base} */ function B() {}" +
"/** @param {A|B} x \n * @return {B|A} */ " +
"function foo(x) { return x; }" +
"/** @constructor */ function Base() {}");
}
public void testDirectPrototypeAssignment1() throws Exception {
testTypes(
"/** @constructor */ function Base() {}" +
"Base.prototype.foo = 3;" +
"/** @constructor \n * @extends {Base} */ function A() {}" +
"A.prototype = new Base();" +
"/** @return {string} */ function foo() { return (new A).foo; }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testDirectPrototypeAssignment2() throws Exception {
// This ensures that we don't attach property 'foo' onto the Base
// instance object.
testTypes(
"/** @constructor */ function Base() {}" +
"/** @constructor \n * @extends {Base} */ function A() {}" +
"A.prototype = new Base();" +
"A.prototype.foo = 3;" +
"/** @return {string} */ function foo() { return (new Base).foo; }");
}
public void testDirectPrototypeAssignment3() throws Exception {
// This verifies that the compiler doesn't crash if the user
// overwrites the prototype of a global variable in a local scope.
testTypes(
"/** @constructor */ var MainWidgetCreator = function() {};" +
"/** @param {Function} ctor */" +
"function createMainWidget(ctor) {" +
" /** @constructor */ function tempCtor() {};" +
" tempCtor.prototype = ctor.prototype;" +
" MainWidgetCreator.superClass_ = ctor.prototype;" +
" MainWidgetCreator.prototype = new tempCtor();" +
"}");
}
public void testGoodImplements1() throws Exception {
testTypes("/** @interface */function Disposable() {}\n" +
"/** @implements {Disposable}\n * @constructor */function f() {}");
}
public void testGoodImplements2() throws Exception {
testTypes("/** @interface */function Base1() {}\n" +
"/** @interface */function Base2() {}\n" +
"/** @constructor\n" +
" * @implements {Base1}\n" +
" * @implements {Base2}\n" +
" */ function derived() {}");
}
public void testGoodImplements3() throws Exception {
testTypes("/** @interface */function Disposable() {}\n" +
"/** @constructor \n @implements {Disposable} */function f() {}");
}
public void testGoodImplements4() throws Exception {
testTypes("var goog = {};" +
"/** @type {!Function} */" +
"goog.abstractMethod = function() {};" +
"/** @interface */\n" +
"goog.Disposable = goog.abstractMethod;" +
"goog.Disposable.prototype.dispose = goog.abstractMethod;" +
"/** @implements {goog.Disposable}\n * @constructor */" +
"goog.SubDisposable = function() {};" +
"/** @inheritDoc */ " +
"goog.SubDisposable.prototype.dispose = function() {};");
}
public void testGoodImplements5() throws Exception {
testTypes(
"/** @interface */\n" +
"goog.Disposable = function() {};" +
"/** @type {Function} */" +
"goog.Disposable.prototype.dispose = function() {};" +
"/** @implements {goog.Disposable}\n * @constructor */" +
"goog.SubDisposable = function() {};" +
"/** @param {number} key \n @override */ " +
"goog.SubDisposable.prototype.dispose = function(key) {};");
}
public void testGoodImplements6() throws Exception {
testTypes(
"var myNullFunction = function() {};" +
"/** @interface */\n" +
"goog.Disposable = function() {};" +
"/** @return {number} */" +
"goog.Disposable.prototype.dispose = myNullFunction;" +
"/** @implements {goog.Disposable}\n * @constructor */" +
"goog.SubDisposable = function() {};" +
"/** @return {number} \n @override */ " +
"goog.SubDisposable.prototype.dispose = function() { return 0; };");
}
public void testGoodImplements7() throws Exception {
testTypes(
"var myNullFunction = function() {};" +
"/** @interface */\n" +
"goog.Disposable = function() {};" +
"/** @return {number} */" +
"goog.Disposable.prototype.dispose = function() {};" +
"/** @implements {goog.Disposable}\n * @constructor */" +
"goog.SubDisposable = function() {};" +
"/** @return {number} \n @override */ " +
"goog.SubDisposable.prototype.dispose = function() { return 0; };");
}
public void testBadImplements1() throws Exception {
testTypes("/** @interface */function Base1() {}\n" +
"/** @interface */function Base2() {}\n" +
"/** @constructor\n" +
" * @implements {nonExistent}\n" +
" * @implements {Base2}\n" +
" */ function derived() {}",
"Bad type annotation. Unknown type nonExistent");
}
public void testBadImplements2() throws Exception {
testTypes("/** @interface */function Disposable() {}\n" +
"/** @implements {Disposable}\n */function f() {}",
"@implements used without @constructor or @interface for f");
}
public void testBadImplements3() throws Exception {
testTypes(
"var goog = {};" +
"/** @type {!Function} */ goog.abstractMethod = function(){};" +
"/** @interface */ var Disposable = goog.abstractMethod;" +
"Disposable.prototype.method = goog.abstractMethod;" +
"/** @implements {Disposable}\n * @constructor */function f() {}",
"property method on interface Disposable is not implemented by type f");
}
public void testBadImplements4() throws Exception {
testTypes("/** @interface */function Disposable() {}\n" +
"/** @implements {Disposable}\n * @interface */function f() {}",
"f cannot implement this type; an interface can only extend, " +
"but not implement interfaces");
}
public void testBadImplements5() throws Exception {
testTypes("/** @interface */function Disposable() {}\n" +
"/** @type {number} */ Disposable.prototype.bar = function() {};",
"assignment to property bar of Disposable.prototype\n" +
"found : function (): undefined\n" +
"required: number");
}
public void testBadImplements6() throws Exception {
testClosureTypesMultipleWarnings(
"/** @interface */function Disposable() {}\n" +
"/** @type {function()} */ Disposable.prototype.bar = 3;",
Lists.newArrayList(
"assignment to property bar of Disposable.prototype\n" +
"found : number\n" +
"required: function (): ?",
"interface members can only be empty property declarations, " +
"empty functions, or goog.abstractMethod"));
}
public void testInterfaceExtends() throws Exception {
testTypes("/** @interface */function A() {}\n" +
"/** @interface \n * @extends {A} */function B() {}\n" +
"/** @constructor\n" +
" * @implements {B}\n" +
" */ function derived() {}");
}
public void testBadInterfaceExtends1() throws Exception {
testTypes("/** @interface \n * @extends {nonExistent} */function A() {}",
"Bad type annotation. Unknown type nonExistent");
}
public void testBadInterfaceExtends2() throws Exception {
testTypes("/** @constructor */function A() {}\n" +
"/** @interface \n * @extends {A} */function B() {}",
"B cannot extend this type; a constructor can only extend objects " +
"and an interface can only extend interfaces");
}
public void testBadInterfaceExtends3() throws Exception {
testTypes("/** @interface */function A() {}\n" +
"/** @constructor \n * @extends {A} */function B() {}",
"B cannot extend this type; a constructor can only extend objects " +
"and an interface can only extend interfaces");
}
public void testBadInterfaceExtends4() throws Exception {
// TODO(user): This should be detected as an error. Even if we enforce
// that A cannot be used in the assignment, we should still detect the
// inheritance chain as invalid.
testTypes("/** @interface */function A() {}\n" +
"/** @constructor */function B() {}\n" +
"B.prototype = A;");
}
public void testBadInterfaceExtends5() throws Exception {
// TODO(user): This should be detected as an error. Even if we enforce
// that A cannot be used in the assignment, we should still detect the
// inheritance chain as invalid.
testTypes("/** @constructor */function A() {}\n" +
"/** @interface */function B() {}\n" +
"B.prototype = A;");
}
public void testBadImplementsAConstructor() throws Exception {
testTypes("/** @constructor */function A() {}\n" +
"/** @constructor \n * @implements {A} */function B() {}",
"can only implement interfaces");
}
public void testBadImplementsNonInterfaceType() throws Exception {
testTypes("/** @constructor \n * @implements {Boolean} */function B() {}",
"can only implement interfaces");
}
public void testBadImplementsNonObjectType() throws Exception {
testTypes("/** @constructor \n * @implements {string} */function S() {}",
"can only implement interfaces");
}
public void testInterfaceAssignment1() throws Exception {
testTypes("/** @interface */var I = function() {};\n" +
"/** @constructor\n@implements {I} */var T = function() {};\n" +
"var t = new T();\n" +
"/** @type {!I} */var i = t;");
}
public void testInterfaceAssignment2() throws Exception {
testTypes("/** @interface */var I = function() {};\n" +
"/** @constructor */var T = function() {};\n" +
"var t = new T();\n" +
"/** @type {!I} */var i = t;",
"initializing variable\n" +
"found : T\n" +
"required: I");
}
public void testInterfaceAssignment3() throws Exception {
testTypes("/** @interface */var I = function() {};\n" +
"/** @constructor\n@implements {I} */var T = function() {};\n" +
"var t = new T();\n" +
"/** @type {I|number} */var i = t;");
}
public void testInterfaceAssignment4() throws Exception {
testTypes("/** @interface */var I1 = function() {};\n" +
"/** @interface */var I2 = function() {};\n" +
"/** @constructor\n@implements {I1} */var T = function() {};\n" +
"var t = new T();\n" +
"/** @type {I1|I2} */var i = t;");
}
public void testInterfaceAssignment5() throws Exception {
testTypes("/** @interface */var I1 = function() {};\n" +
"/** @interface */var I2 = function() {};\n" +
"/** @constructor\n@implements {I1}\n@implements {I2}*/" +
"var T = function() {};\n" +
"var t = new T();\n" +
"/** @type {I1} */var i1 = t;\n" +
"/** @type {I2} */var i2 = t;\n");
}
public void testInterfaceAssignment6() throws Exception {
testTypes("/** @interface */var I1 = function() {};\n" +
"/** @interface */var I2 = function() {};\n" +
"/** @constructor\n@implements {I1} */var T = function() {};\n" +
"/** @type {!I1} */var i1 = new T();\n" +
"/** @type {!I2} */var i2 = i1;\n",
"initializing variable\n" +
"found : I1\n" +
"required: I2");
}
public void testInterfaceAssignment7() throws Exception {
testTypes("/** @interface */var I1 = function() {};\n" +
"/** @interface\n@extends {I1}*/var I2 = function() {};\n" +
"/** @constructor\n@implements {I2}*/var T = function() {};\n" +
"var t = new T();\n" +
"/** @type {I1} */var i1 = t;\n" +
"/** @type {I2} */var i2 = t;\n" +
"i1 = i2;\n");
}
public void testInterfaceAssignment8() throws Exception {
testTypes("/** @interface */var I = function() {};\n" +
"/** @type {I} */var i;\n" +
"/** @type {Object} */var o = i;\n" +
"new Object().prototype = i.prototype;");
}
public void testInterfaceAssignment9() throws Exception {
testTypes("/** @interface */var I = function() {};\n" +
"/** @return {I?} */function f() { return null; }\n" +
"/** @type {!I} */var i = f();\n",
"initializing variable\n" +
"found : (I|null)\n" +
"required: I");
}
public void testInterfaceAssignment10() throws Exception {
testTypes("/** @interface */var I1 = function() {};\n" +
"/** @interface */var I2 = function() {};\n" +
"/** @constructor\n@implements {I2} */var T = function() {};\n" +
"/** @return {!I1|!I2} */function f() { return new T(); }\n" +
"/** @type {!I1} */var i1 = f();\n",
"initializing variable\n" +
"found : (I1|I2)\n" +
"required: I1");
}
public void testInterfaceAssignment11() throws Exception {
testTypes("/** @interface */var I1 = function() {};\n" +
"/** @interface */var I2 = function() {};\n" +
"/** @constructor */var T = function() {};\n" +
"/** @return {!I1|!I2|!T} */function f() { return new T(); }\n" +
"/** @type {!I1} */var i1 = f();\n",
"initializing variable\n" +
"found : (I1|I2|T)\n" +
"required: I1");
}
public void testInterfaceAssignment12() throws Exception {
testTypes("/** @interface */var I = function() {};\n" +
"/** @constructor\n@implements{I}*/var T1 = function() {};\n" +
"/** @constructor\n@extends {T1}*/var T2 = function() {};\n" +
"/** @return {I} */function f() { return new T2(); }");
}
public void testInterfaceAssignment13() throws Exception {
testTypes("/** @interface */var I = function() {};\n" +
"/** @constructor\n@implements {I}*/var T = function() {};\n" +
"/** @constructor */function Super() {};\n" +
"/** @return {I} */Super.prototype.foo = " +
"function() { return new T(); };\n" +
"/** @constructor\n@extends {Super} */function Sub() {}\n" +
"/** @override\n@return {T} */Sub.prototype.foo = " +
"function() { return new T(); };\n");
}
public void testGetprop1() throws Exception {
testTypes("/** @return {void}*/function foo(){foo().bar;}",
"No properties on this expression\n" +
"found : undefined\n" +
"required: Object");
}
public void testGetprop2() throws Exception {
testTypes("var x = null; x.alert();",
"No properties on this expression\n" +
"found : null\n" +
"required: Object");
}
public void testGetprop3() throws Exception {
testTypes(
"/** @constructor */ " +
"function Foo() { /** @type {?Object} */ this.x = null; }" +
"Foo.prototype.initX = function() { this.x = {foo: 1}; };" +
"Foo.prototype.bar = function() {" +
" if (this.x == null) { this.initX(); alert(this.x.foo); }" +
"};");
}
public void testArrayAccess1() throws Exception {
testTypes("var a = []; var b = a['hi'];");
}
public void testArrayAccess2() throws Exception {
testTypes("var a = []; var b = a[[1,2]];",
"array access\n" +
"found : Array\n" +
"required: number");
}
public void testArrayAccess3() throws Exception {
testTypes("var bar = [];" +
"/** @return {void} */function baz(){};" +
"var foo = bar[baz()];",
"array access\n" +
"found : undefined\n" +
"required: number");
}
public void testArrayAccess4() throws Exception {
testTypes("/**@return {!Array}*/function foo(){};var bar = foo()[foo()];",
"array access\n" +
"found : Array\n" +
"required: number");
}
public void testArrayAccess6() throws Exception {
testTypes("var bar = null[1];",
"only arrays or objects can be accessed\n" +
"found : null\n" +
"required: Object");
}
public void testArrayAccess7() throws Exception {
testTypes("var bar = void 0; bar[0];",
"only arrays or objects can be accessed\n" +
"found : undefined\n" +
"required: Object");
}
public void testArrayAccess8() throws Exception {
// Verifies that we don't emit two warnings, because
// the var has been dereferenced after the first one.
testTypes("var bar = void 0; bar[0]; bar[1];",
"only arrays or objects can be accessed\n" +
"found : undefined\n" +
"required: Object");
}
public void testArrayAccess9() throws Exception {
testTypes("/** @return {?Array} */ function f() { return []; }" +
"f()[{}]",
"array access\n" +
"found : {}\n" +
"required: number");
}
public void testPropAccess() throws Exception {
testTypes("/** @param {*} x */var f = function(x) {\n" +
"var o = String(x);\n" +
"if (typeof o['a'] != 'undefined') { return o['a']; }\n" +
"return null;\n" +
"};");
}
public void testPropAccess2() throws Exception {
testTypes("var bar = void 0; bar.baz;",
"No properties on this expression\n" +
"found : undefined\n" +
"required: Object");
}
public void testPropAccess3() throws Exception {
// Verifies that we don't emit two warnings, because
// the var has been dereferenced after the first one.
testTypes("var bar = void 0; bar.baz; bar.bax;",
"No properties on this expression\n" +
"found : undefined\n" +
"required: Object");
}
public void testPropAccess4() throws Exception {
testTypes("/** @param {*} x */ function f(x) { return x['hi']; }");
}
public void testSwitchCase1() throws Exception {
testTypes("/**@type number*/var a;" +
"/**@type string*/var b;" +
"switch(a){case b:;}",
"case expression doesn't match switch\n" +
"found : string\n" +
"required: number");
}
public void testSwitchCase2() throws Exception {
testTypes("var a = null; switch (typeof a) { case 'foo': }");
}
public void testVar1() throws Exception {
TypeCheckResult p =
parseAndTypeCheckWithScope("/** @type {(string,null)} */var a = null");
assertEquals(createUnionType(STRING_TYPE, NULL_TYPE),
p.scope.getVar("a").getType());
}
public void testVar2() throws Exception {
testTypes("/** @type {Function} */ var a = function(){}");
}
public void testVar3() throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope("var a = 3;");
assertEquals(NUMBER_TYPE, p.scope.getVar("a").getType());
}
public void testVar4() throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope(
"var a = 3; a = 'string';");
assertEquals(createUnionType(STRING_TYPE, NUMBER_TYPE),
p.scope.getVar("a").getType());
}
public void testVar5() throws Exception {
testTypes("var goog = {};" +
"/** @type string */goog.foo = 'hello';" +
"/** @type number */var a = goog.foo;",
"initializing variable\n" +
"found : string\n" +
"required: number");
}
public void testVar6() throws Exception {
testTypes(
"function f() {" +
" return function() {" +
" /** @type {!Date} */" +
" var a = 7;" +
" };" +
"}",
"initializing variable\n" +
"found : number\n" +
"required: Date");
}
public void testVar7() throws Exception {
testTypes("/** @type number */var a, b;",
"declaration of multiple variables with shared type information");
}
public void testVar8() throws Exception {
testTypes("var a, b;");
}
public void testVar9() throws Exception {
testTypes("/** @enum */var a;",
"enum initializer must be an object literal or an enum");
}
public void testVar10() throws Exception {
testTypes("/** @type !Number */var foo = 'abc';",
"initializing variable\n" +
"found : string\n" +
"required: Number");
}
public void testVar11() throws Exception {
testTypes("var /** @type !Date */foo = 'abc';",
"initializing variable\n" +
"found : string\n" +
"required: Date");
}
public void testVar12() throws Exception {
testTypes("var /** @type !Date */foo = 'abc', " +
"/** @type !RegExp */bar = 5;",
new String[] {
"initializing variable\n" +
"found : string\n" +
"required: Date",
"initializing variable\n" +
"found : number\n" +
"required: RegExp"});
}
public void testVar13() throws Exception {
// this caused an NPE
testTypes("var /** @type number */a,a;");
}
public void testVar14() throws Exception {
testTypes("/** @return {number} */ function f() { var x; return x; }",
"inconsistent return type\n" +
"found : undefined\n" +
"required: number");
}
public void testVar15() throws Exception {
testTypes("/** @return {number} */" +
"function f() { var x = x || {}; return x; }",
"inconsistent return type\n" +
"found : {}\n" +
"required: number");
}
public void testAssign1() throws Exception {
testTypes("var goog = {};" +
"/** @type number */goog.foo = 'hello';",
"assignment to property foo of goog\n" +
"found : string\n" +
"required: number");
}
public void testAssign2() throws Exception {
testTypes("var goog = {};" +
"/** @type number */goog.foo = 3;" +
"goog.foo = 'hello';",
"assignment to property foo of goog\n" +
"found : string\n" +
"required: number");
}
public void testAssign3() throws Exception {
testTypes("var goog = {};" +
"/** @type number */goog.foo = 3;" +
"goog.foo = 4;");
}
public void testAssign4() throws Exception {
testTypes("var goog = {};" +
"goog.foo = 3;" +
"goog.foo = 'hello';");
}
public void testAssignInference() throws Exception {
testTypes(
"/**" +
" * @param {Array} x" +
" * @return {number}" +
" */" +
"function f(x) {" +
" var y = null;" +
" y = x[0];" +
" if (y == null) { return 4; } else { return 6; }" +
"}");
}
public void testOr1() throws Exception {
testTypes("/** @type number */var a;" +
"/** @type number */var b;" +
"a + b || undefined;");
}
public void testOr2() throws Exception {
testTypes("/** @type number */var a;" +
"/** @type number */var b;" +
"/** @type number */var c = a + b || undefined;",
"initializing variable\n" +
"found : (number|undefined)\n" +
"required: number");
}
public void testOr3() throws Exception {
testTypes("/** @type {(number, undefined)} */var a;" +
"/** @type number */var c = a || 3;");
}
/**
* Test that type inference continues with the right side,
* when no short-circuiting is possible.
* See bugid 1205387 for more details.
*/
public void testOr4() throws Exception {
testTypes("/**@type {number} */var x;x=null || \"a\";",
"assignment\n" +
"found : string\n" +
"required: number");
}
/**
* @see #testOr4()
*/
public void testOr5() throws Exception {
testTypes("/**@type {number} */var x;x=undefined || \"a\";",
"assignment\n" +
"found : string\n" +
"required: number");
}
public void testAnd1() throws Exception {
testTypes("/** @type number */var a;" +
"/** @type number */var b;" +
"a + b && undefined;");
}
public void testAnd2() throws Exception {
testTypes("/** @type number */var a;" +
"/** @type number */var b;" +
"/** @type number */var c = a + b && undefined;",
"initializing variable\n" +
"found : (number|undefined)\n" +
"required: number");
}
public void testAnd3() throws Exception {
testTypes("/** @type {(!Array, undefined)} */var a;" +
"/** @type number */var c = a && undefined;",
"initializing variable\n" +
"found : undefined\n" +
"required: number");
}
public void testAnd4() throws Exception {
testTypes("/** @param {number} x */function f(x){};\n" +
"/** @type null */var x; /** @type {number?} */var y;\n" +
"if (x && y) { f(y) }");
}
public void testAnd5() throws Exception {
testTypes("/** @param {number} x\n@param {string} y*/function f(x,y){};\n" +
"/** @type {number?} */var x; /** @type {string?} */var y;\n" +
"if (x && y) { f(x, y) }");
}
public void testAnd6() throws Exception {
testTypes("/** @param {number} x */function f(x){};\n" +
"/** @type {number|undefined} */var x;\n" +
"if (x && f(x)) { f(x) }");
}
public void testAnd7() throws Exception {
// TODO(user): a deterministic warning should be generated for this
// case since x && x is always false. The implementation of this requires
// a more precise handling of a null value within a variable's type.
// Currently, a null value defaults to ? which passes every check.
testTypes("/** @type null */var x; if (x && x) {}");
}
public void testHook() throws Exception {
testTypes("/**@return {void}*/function foo(){ var x=foo()?a:b; }");
}
public void testHookRestrictsType1() throws Exception {
testTypes("/** @return {(string,null)} */" +
"function f() { return null;}" +
"/** @type {(string,null)} */ var a = f();" +
"/** @type string */" +
"var b = a ? a : 'default';");
}
public void testHookRestrictsType2() throws Exception {
testTypes("/** @type {String} */" +
"var a = null;" +
"/** @type null */" +
"var b = a ? null : a;");
}
public void testHookRestrictsType3() throws Exception {
testTypes("/** @type {String} */" +
"var a;" +
"/** @type null */" +
"var b = (!a) ? a : null;");
}
public void testHookRestrictsType4() throws Exception {
testTypes("/** @type {(boolean,undefined)} */" +
"var a;" +
"/** @type boolean */" +
"var b = a != null ? a : true;");
}
public void testHookRestrictsType5() throws Exception {
testTypes("/** @type {(boolean,undefined)} */" +
"var a;" +
"/** @type {(undefined)} */" +
"var b = a == null ? a : undefined;");
}
public void testHookRestrictsType6() throws Exception {
testTypes("/** @type {(number,null,undefined)} */" +
"var a;" +
"/** @type {number} */" +
"var b = a == null ? 5 : a;");
}
public void testHookRestrictsType7() throws Exception {
testTypes("/** @type {(number,null,undefined)} */" +
"var a;" +
"/** @type {number} */" +
"var b = a == undefined ? 5 : a;");
}
public void testWhileRestrictsType1() throws Exception {
testTypes("/** @param {null} x */ function g(x) {}" +
"/** @param {number?} x */\n" +
"function f(x) {\n" +
"while (x) {\n" +
"if (g(x)) { x = 1; }\n" +
"x = x-1;\n}\n}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : number\n" +
"required: null");
}
public void testWhileRestrictsType2() throws Exception {
testTypes("/** @param {number?} x\n@return {number}*/\n" +
"function f(x) {\n/** @type {number} */var y = 0;" +
"while (x) {\n" +
"y = x;\n" +
"x = x-1;\n}\n" +
"return y;}");
}
public void testHigherOrderFunctions1() throws Exception {
testTypes(
"/** @type {function(number)} */var f;" +
"f(true);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testHigherOrderFunctions2() throws Exception {
testTypes(
"/** @type {function():!Date} */var f;" +
"/** @type boolean */var a = f();",
"initializing variable\n" +
"found : Date\n" +
"required: boolean");
}
public void testHigherOrderFunctions3() throws Exception {
testTypes(
"/** @type {function(this:Error):Date} */var f; new f",
"cannot instantiate non-constructor");
}
public void testHigherOrderFunctions4() throws Exception {
testTypes(
"/** @type {function(this:Error,...[number]):Date} */var f; new f",
"cannot instantiate non-constructor");
}
public void testHigherOrderFunctions5() throws Exception {
testTypes(
"/** @param {number} x */ function g(x) {}" +
"/** @type {function(new:Error,...[number]):Date} */ var f;" +
"g(new f());",
"actual parameter 1 of g does not match formal parameter\n" +
"found : Error\n" +
"required: number");
}
public void testConstructorAlias1() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {};" +
"/** @type {number} */ Foo.prototype.bar = 3;" +
"/** @constructor */ var FooAlias = Foo;" +
"/** @return {string} */ function foo() { " +
" return (new FooAlias()).bar; }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testConstructorAlias2() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {};" +
"/** @constructor */ var FooAlias = Foo;" +
"/** @type {number} */ FooAlias.prototype.bar = 3;" +
"/** @return {string} */ function foo() { " +
" return (new Foo()).bar; }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testConstructorAlias3() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {};" +
"/** @type {number} */ Foo.prototype.bar = 3;" +
"/** @constructor */ var FooAlias = Foo;" +
"/** @return {string} */ function foo() { " +
" return (new FooAlias()).bar; }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testConstructorAlias4() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {};" +
"var FooAlias = Foo;" +
"/** @type {number} */ FooAlias.prototype.bar = 3;" +
"/** @return {string} */ function foo() { " +
" return (new Foo()).bar; }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testConstructorAlias5() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {};" +
"/** @constructor */ var FooAlias = Foo;" +
"/** @return {FooAlias} */ function foo() { " +
" return new Foo(); }");
}
public void testConstructorAlias6() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {};" +
"/** @constructor */ var FooAlias = Foo;" +
"/** @return {Foo} */ function foo() { " +
" return new FooAlias(); }");
}
public void testConstructorAlias7() throws Exception {
testTypes(
"var goog = {};" +
"/** @constructor */ goog.Foo = function() {};" +
"/** @constructor */ goog.FooAlias = goog.Foo;" +
"/** @return {number} */ function foo() { " +
" return new goog.FooAlias(); }",
"inconsistent return type\n" +
"found : goog.Foo\n" +
"required: number");
}
public void testConstructorAlias8() throws Exception {
testTypes(
"var goog = {};" +
"/**\n * @param {number} x \n * @constructor */ " +
"goog.Foo = function(x) {};" +
"/**\n * @param {number} x \n * @constructor */ " +
"goog.FooAlias = goog.Foo;" +
"/** @return {number} */ function foo() { " +
" return new goog.FooAlias(1); }",
"inconsistent return type\n" +
"found : goog.Foo\n" +
"required: number");
}
public void testConstructorAlias9() throws Exception {
testTypes(
"var goog = {};" +
"/**\n * @param {number} x \n * @constructor */ " +
"goog.Foo = function(x) {};" +
"/** @constructor */ goog.FooAlias = goog.Foo;" +
"/** @return {number} */ function foo() { " +
" return new goog.FooAlias(1); }",
"inconsistent return type\n" +
"found : goog.Foo\n" +
"required: number");
}
public void testConstructorAlias10() throws Exception {
testTypes(
"/**\n * @param {number} x \n * @constructor */ " +
"var Foo = function(x) {};" +
"/** @constructor */ var FooAlias = Foo;" +
"/** @return {number} */ function foo() { " +
" return new FooAlias(1); }",
"inconsistent return type\n" +
"found : Foo\n" +
"required: number");
}
public void testClosure1() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {string|undefined} */var a;" +
"/** @type string */" +
"var b = goog.isDef(a) ? a : 'default';",
null);
}
public void testClosure2() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {string?} */var a;" +
"/** @type string */" +
"var b = goog.isNull(a) ? 'default' : a;",
null);
}
public void testClosure3() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {string|null|undefined} */var a;" +
"/** @type string */" +
"var b = goog.isDefAndNotNull(a) ? a : 'default';",
null);
}
public void testClosure4() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {string|undefined} */var a;" +
"/** @type string */" +
"var b = !goog.isDef(a) ? 'default' : a;",
null);
}
public void testClosure5() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {string?} */var a;" +
"/** @type string */" +
"var b = !goog.isNull(a) ? a : 'default';",
null);
}
public void testClosure6() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {string|null|undefined} */var a;" +
"/** @type string */" +
"var b = !goog.isDefAndNotNull(a) ? 'default' : a;",
null);
}
public void testClosure7() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {string|null|undefined} */ var a = foo();" +
"/** @type {number} */" +
"var b = goog.asserts.assert(a);",
"initializing variable\n" +
"found : string\n" +
"required: number");
}
public void testReturn1() throws Exception {
testTypes("/**@return {void}*/function foo(){ return 3; }",
"inconsistent return type\n" +
"found : number\n" +
"required: undefined");
}
public void testReturn2() throws Exception {
testTypes("/**@return {!Number}*/function foo(){ return; }",
"inconsistent return type\n" +
"found : undefined\n" +
"required: Number");
}
public void testReturn3() throws Exception {
testTypes("/**@return {!Number}*/function foo(){ return 'abc'; }",
"inconsistent return type\n" +
"found : string\n" +
"required: Number");
}
public void testReturn4() throws Exception {
testTypes("/**@return {!Number}\n*/\n function a(){return new Array();}",
"inconsistent return type\n" +
"found : Array\n" +
"required: Number");
}
public void testReturn5() throws Exception {
testTypes("/** @param {number} n\n" +
"@constructor */function n(n){return};");
}
public void testReturn6() throws Exception {
testTypes(
"/** @param {number} opt_a\n@return {string} */" +
"function a(opt_a) { return opt_a }",
"inconsistent return type\n" +
"found : (number|undefined)\n" +
"required: string");
}
public void testReturn7() throws Exception {
testTypes("/** @constructor */var A = function() {};\n" +
"/** @constructor */var B = function() {};\n" +
"/** @return {!B} */A.f = function() { return 1; };",
"inconsistent return type\n" +
"found : number\n" +
"required: B");
}
public void testReturn8() throws Exception {
testTypes("/** @constructor */var A = function() {};\n" +
"/** @constructor */var B = function() {};\n" +
"/** @return {!B} */A.prototype.f = function() { return 1; };",
"inconsistent return type\n" +
"found : number\n" +
"required: B");
}
public void testInferredReturn1() throws Exception {
testTypes(
"function f() {} /** @param {number} x */ function g(x) {}" +
"g(f());",
"actual parameter 1 of g does not match formal parameter\n" +
"found : undefined\n" +
"required: number");
}
public void testInferredReturn2() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() {}; " +
"/** @param {number} x */ function g(x) {}" +
"g((new Foo()).bar());",
"actual parameter 1 of g does not match formal parameter\n" +
"found : undefined\n" +
"required: number");
}
public void testInferredReturn3() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() {}; " +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @return {number} \n * @override */ " +
"SubFoo.prototype.bar = function() { return 3; }; ",
"mismatch of the bar property type and the type of the property " +
"it overrides from superclass Foo\n" +
"original: function (this:Foo): undefined\n" +
"override: function (this:SubFoo): number");
}
public void testInferredReturn4() throws Exception {
// By design, this throws a warning. if you want global x to be
// defined to some other type of function, then you need to declare it
// as a greater type.
testTypes(
"var x = function() {};" +
"x = /** @type {function(): number} */ (function() { return 3; });",
"assignment\n" +
"found : function (): number\n" +
"required: function (): undefined");
}
public void testInferredReturn5() throws Exception {
// If x is local, then the function type is not declared.
testTypes(
"/** @return {string} */" +
"function f() {" +
" var x = function() {};" +
" x = /** @type {function(): number} */ (function() { return 3; });" +
" return x();" +
"}",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testInferredReturn6() throws Exception {
testTypes(
"/** @return {string} */" +
"function f() {" +
" var x = function() {};" +
" if (f()) " +
" x = /** @type {function(): number} */ " +
" (function() { return 3; });" +
" return x();" +
"}",
"inconsistent return type\n" +
"found : (number|undefined)\n" +
"required: string");
}
public void testInferredReturn7() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @param {number} x */ Foo.prototype.bar = function(x) {};" +
"Foo.prototype.bar = function(x) { return 3; };",
"inconsistent return type\n" +
"found : number\n" +
"required: undefined");
}
public void testInferredReturn8() throws Exception {
reportMissingOverrides = CheckLevel.OFF;
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @param {number} x */ Foo.prototype.bar = function(x) {};" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @param {number} x */ SubFoo.prototype.bar = " +
" function(x) { return 3; }",
"inconsistent return type\n" +
"found : number\n" +
"required: undefined");
}
public void testInferredParam1() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @param {number} x */ Foo.prototype.bar = function(x) {};" +
"/** @param {string} x */ function f(x) {}" +
"Foo.prototype.bar = function(y) { f(y); };",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testInferredParam2() throws Exception {
reportMissingOverrides = CheckLevel.OFF;
testTypes(
"/** @param {string} x */ function f(x) {}" +
"/** @constructor */ function Foo() {}" +
"/** @param {number} x */ Foo.prototype.bar = function(x) {};" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @return {void} */ SubFoo.prototype.bar = " +
" function(x) { f(x); }",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testInferredParam3() throws Exception {
reportMissingOverrides = CheckLevel.OFF;
testTypes(
"/** @param {string} x */ function f(x) {}" +
"/** @constructor */ function Foo() {}" +
"/** @param {number=} x */ Foo.prototype.bar = function(x) {};" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @return {void} */ SubFoo.prototype.bar = " +
" function(x) { f(x); }; (new SubFoo()).bar();",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (number|undefined)\n" +
"required: string");
}
public void testInferredParam4() throws Exception {
reportMissingOverrides = CheckLevel.OFF;
testTypes(
"/** @param {string} x */ function f(x) {}" +
"/** @constructor */ function Foo() {}" +
"/** @param {...number} x */ Foo.prototype.bar = function(x) {};" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @return {void} */ SubFoo.prototype.bar = " +
" function(x) { f(x); }; (new SubFoo()).bar();",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (number|undefined)\n" +
"required: string");
}
public void testInferredParam5() throws Exception {
reportMissingOverrides = CheckLevel.OFF;
testTypes(
"/** @param {string} x */ function f(x) {}" +
"/** @constructor */ function Foo() {}" +
"/** @param {...number} x */ Foo.prototype.bar = function(x) {};" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @param {number=} x \n * @param {...number} y */ " +
"SubFoo.prototype.bar = " +
" function(x, y) { f(x); }; (new SubFoo()).bar();",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (number|undefined)\n" +
"required: string");
}
public void testInferredParam6() throws Exception {
reportMissingOverrides = CheckLevel.OFF;
testTypes(
"/** @param {string} x */ function f(x) {}" +
"/** @constructor */ function Foo() {}" +
"/** @param {number=} x */ Foo.prototype.bar = function(x) {};" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @param {number=} x \n * @param {number=} y */ " +
"SubFoo.prototype.bar = " +
" function(x, y) { f(y); };",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (number|undefined)\n" +
"required: string");
}
public void testOverriddenParams1() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @param {...?} var_args */" +
"Foo.prototype.bar = function(var_args) {};" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/**\n" +
" * @param {number} x\n" +
" * @override\n" +
" */" +
"SubFoo.prototype.bar = function(x) {};");
}
public void testOverriddenParams2() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @type {function(...[?])} */" +
"Foo.prototype.bar = function(var_args) {};" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/**\n" +
" * @type {function(number)}\n" +
" * @override\n" +
" */" +
"SubFoo.prototype.bar = function(x) {};");
}
public void testOverriddenParams3() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @param {...number} var_args */" +
"Foo.prototype.bar = function(var_args) { };" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/**\n" +
" * @param {number} x\n" +
" * @override\n" +
" */" +
"SubFoo.prototype.bar = function(x) {};",
"mismatch of the bar property type and the type of the " +
"property it overrides from superclass Foo\n" +
"original: function (this:Foo, ...[number]): undefined\n" +
"override: function (this:SubFoo, number): undefined");
}
public void testOverriddenParams4() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @type {function(...[number])} */" +
"Foo.prototype.bar = function(var_args) {};" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/**\n" +
" * @type {function(number)}\n" +
" * @override\n" +
" */" +
"SubFoo.prototype.bar = function(x) {};",
"mismatch of the bar property type and the type of the " +
"property it overrides from superclass Foo\n" +
"original: function (...[number]): ?\n" +
"override: function (number): ?");
}
public void testOverriddenParams5() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @param {number} x */" +
"Foo.prototype.bar = function(x) { };" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/**\n" +
" * @override\n" +
" */" +
"SubFoo.prototype.bar = function() {};" +
"(new SubFoo()).bar();");
}
public void testOverriddenParams6() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @param {number} x */" +
"Foo.prototype.bar = function(x) { };" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/**\n" +
" * @override\n" +
" */" +
"SubFoo.prototype.bar = function() {};" +
"(new SubFoo()).bar(true);",
"actual parameter 1 of SubFoo.prototype.bar " +
"does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testOverriddenReturn1() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @return {Object} */ Foo.prototype.bar = " +
" function() { return {}; };" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @return {SubFoo}\n * @override */ SubFoo.prototype.bar = " +
" function() { return new Foo(); }",
"inconsistent return type\n" +
"found : Foo\n" +
"required: (SubFoo|null)");
}
public void testOverriddenReturn2() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @return {SubFoo} */ Foo.prototype.bar = " +
" function() { return new SubFoo(); };" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @return {Foo} x\n * @override */ SubFoo.prototype.bar = " +
" function() { return new SubFoo(); }",
"mismatch of the bar property type and the type of the " +
"property it overrides from superclass Foo\n" +
"original: function (this:Foo): (SubFoo|null)\n" +
"override: function (this:SubFoo): (Foo|null)");
}
public void testThis1() throws Exception {
testTypes("var goog = {};" +
"/** @constructor */goog.A = function(){};" +
"/** @return {number} */" +
"goog.A.prototype.n = function() { return this };",
"inconsistent return type\n" +
"found : goog.A\n" +
"required: number");
}
public void testOverriddenProperty1() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @type {Object} */" +
"Foo.prototype.bar = {};" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/**\n" +
" * @type {Array}\n" +
" * @override\n" +
" */" +
"SubFoo.prototype.bar = [];");
}
public void testOverriddenProperty2() throws Exception {
testTypes(
"/** @constructor */ function Foo() {" +
" /** @type {Object} */" +
" this.bar = {};" +
"}" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/**\n" +
" * @type {Array}\n" +
" * @override\n" +
" */" +
"SubFoo.prototype.bar = [];");
}
public void testThis2() throws Exception {
testTypes("var goog = {};" +
"/** @constructor */goog.A = function(){" +
" this.foo = null;" +
"};" +
"/** @return {number} */" +
"goog.A.prototype.n = function() { return this.foo };",
"inconsistent return type\n" +
"found : null\n" +
"required: number");
}
public void testThis3() throws Exception {
testTypes("var goog = {};" +
"/** @constructor */goog.A = function(){" +
" this.foo = null;" +
" this.foo = 5;" +
"};");
}
public void testThis4() throws Exception {
testTypes("var goog = {};" +
"/** @constructor */goog.A = function(){" +
" /** @type {string?} */this.foo = null;" +
"};" +
"/** @return {number} */goog.A.prototype.n = function() {" +
" return this.foo };",
"inconsistent return type\n" +
"found : (null|string)\n" +
"required: number");
}
public void testThis5() throws Exception {
testTypes("/** @this Date\n@return {number}*/function h() { return this }",
"inconsistent return type\n" +
"found : Date\n" +
"required: number");
}
public void testThis6() throws Exception {
testTypes("var goog = {};" +
"/** @constructor\n@return {!Date} */" +
"goog.A = function(){ return this };",
"inconsistent return type\n" +
"found : goog.A\n" +
"required: Date");
}
public void testThis7() throws Exception {
testTypes("/** @constructor */function A(){};" +
"/** @return {number} */A.prototype.n = function() { return this };",
"inconsistent return type\n" +
"found : A\n" +
"required: number");
}
public void testThis8() throws Exception {
testTypes("/** @constructor */function A(){" +
" /** @type {string?} */this.foo = null;" +
"};" +
"/** @return {number} */A.prototype.n = function() {" +
" return this.foo };",
"inconsistent return type\n" +
"found : (null|string)\n" +
"required: number");
}
public void testThis9() throws Exception {
// In A.bar, the type of {@code this} is unknown.
testTypes("/** @constructor */function A(){};" +
"A.prototype.foo = 3;" +
"/** @return {string} */ A.bar = function() { return this.foo; };");
}
public void testThis10() throws Exception {
// In A.bar, the type of {@code this} is inferred from the @this tag.
testTypes("/** @constructor */function A(){};" +
"A.prototype.foo = 3;" +
"/** @this {A}\n@return {string} */" +
"A.bar = function() { return this.foo; };",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testThis11() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"/** @constructor */ function Ctor() {" +
" /** @this {Date} */" +
" this.method = function() {" +
" f(this);" +
" };" +
"}",
"actual parameter 1 of f does not match formal parameter\n" +
"found : Date\n" +
"required: number");
}
public void testThis12() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"/** @constructor */ function Ctor() {}" +
"Ctor.prototype['method'] = function() {" +
" f(this);" +
"}",
"actual parameter 1 of f does not match formal parameter\n" +
"found : Ctor\n" +
"required: number");
}
public void testThis13() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"/** @constructor */ function Ctor() {}" +
"Ctor.prototype = {" +
" method: function() {" +
" f(this);" +
" }" +
"};",
"actual parameter 1 of f does not match formal parameter\n" +
"found : Ctor\n" +
"required: number");
}
public void testThis14() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"f(this.Object);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : function (new:Object, *=): ?\n" +
"required: number");
}
public void testThisTypeOfFunction1() throws Exception {
testTypes(
"/** @type {function(this:Object)} */ function f() {}" +
"f();");
}
public void testThisTypeOfFunction2() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** @type {function(this:F)} */ function f() {}" +
"f();",
"\"function (this:F): ?\" must be called with a \"this\" type");
}
public void testThisTypeOfFunction3() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"F.prototype.bar = function() {};" +
"var f = (new F()).bar; f();",
"\"function (this:F): undefined\" must be called with a \"this\" type");
}
public void testThisTypeOfFunction4() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"F.prototype.moveTo = function(x, y) {};" +
"F.prototype.lineTo = function(x, y) {};" +
"function demo() {" +
" var path = new F();" +
" var points = [[1,1], [2,2]];" +
" for (var i = 0; i < points.length; i++) {" +
" (i == 0 ? path.moveTo : path.lineTo)(" +
" points[i][0], points[i][1]);" +
" }" +
"}",
"\"function (this:F, ?, ?): undefined\" " +
"must be called with a \"this\" type");
}
public void testGlobalThis1() throws Exception {
testTypes("/** @constructor */ function Window() {}" +
"/** @param {string} msg */ " +
"Window.prototype.alert = function(msg) {};" +
"this.alert(3);",
"actual parameter 1 of Window.prototype.alert " +
"does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testGlobalThis2() throws Exception {
// this.alert = 3 doesn't count as a declaration, so this isn't a warning.
testTypes("/** @constructor */ function Bindow() {}" +
"/** @param {string} msg */ " +
"Bindow.prototype.alert = function(msg) {};" +
"this.alert = 3;" +
"(new Bindow()).alert(this.alert)");
}
public void testGlobalThis2b() throws Exception {
testTypes("/** @constructor */ function Bindow() {}" +
"/** @param {string} msg */ " +
"Bindow.prototype.alert = function(msg) {};" +
"/** @return {number} */ this.alert = function() { return 3; };" +
"(new Bindow()).alert(this.alert())",
"actual parameter 1 of Bindow.prototype.alert " +
"does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testGlobalThis3() throws Exception {
testTypes(
"/** @param {string} msg */ " +
"function alert(msg) {};" +
"this.alert(3);",
"actual parameter 1 of global this.alert " +
"does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testGlobalThis4() throws Exception {
testTypes(
"/** @param {string} msg */ " +
"var alert = function(msg) {};" +
"this.alert(3);",
"actual parameter 1 of global this.alert " +
"does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testGlobalThis5() throws Exception {
testTypes(
"function f() {" +
" /** @param {string} msg */ " +
" var alert = function(msg) {};" +
"}" +
"this.alert(3);",
"Property alert never defined on global this");
}
public void testGlobalThis6() throws Exception {
testTypes(
"/** @param {string} msg */ " +
"var alert = function(msg) {};" +
"var x = 3;" +
"x = 'msg';" +
"this.alert(this.x);");
}
public void testGlobalThis7() throws Exception {
testTypes(
"/** @constructor */ function Window() {}" +
"/** @param {Window} msg */ " +
"var foo = function(msg) {};" +
"foo(this);");
}
public void testGlobalThis8() throws Exception {
testTypes(
"/** @constructor */ function Window() {}" +
"/** @param {number} msg */ " +
"var foo = function(msg) {};" +
"foo(this);",
"actual parameter 1 of foo does not match formal parameter\n" +
"found : global this\n" +
"required: number");
}
public void testGlobalThis9() throws Exception {
testTypes(
// Window is not marked as a constructor, so the
// inheritance doesn't happen.
"function Window() {}" +
"Window.prototype.alert = function() {};" +
"this.alert();",
"Property alert never defined on global this");
}
public void testControlFlowRestrictsType1() throws Exception {
testTypes("/** @return {String?} */ function f() { return null; }" +
"/** @type {String?} */ var a = f();" +
"/** @type String */ var b = new String('foo');" +
"/** @type null */ var c = null;" +
"if (a) {" +
" b = a;" +
"} else {" +
" c = a;" +
"}");
}
public void testControlFlowRestrictsType2() throws Exception {
testTypes("/** @return {(string,null)} */ function f() { return null; }" +
"/** @type {(string,null)} */ var a = f();" +
"/** @type string */ var b = 'foo';" +
"/** @type null */ var c = null;" +
"if (a) {" +
" b = a;" +
"} else {" +
" c = a;" +
"}",
"assignment\n" +
"found : (null|string)\n" +
"required: null");
}
public void testControlFlowRestrictsType3() throws Exception {
testTypes("/** @type {(string,void)} */" +
"var a;" +
"/** @type string */" +
"var b = 'foo';" +
"if (a) {" +
" b = a;" +
"}");
}
public void testControlFlowRestrictsType4() throws Exception {
testTypes("/** @param {string} a */ function f(a){}" +
"/** @type {(string,undefined)} */ var a;" +
"a && f(a);");
}
public void testControlFlowRestrictsType5() throws Exception {
testTypes("/** @param {undefined} a */ function f(a){}" +
"/** @type {(!Array,undefined)} */ var a;" +
"a || f(a);");
}
public void testControlFlowRestrictsType6() throws Exception {
testTypes("/** @param {undefined} x */ function f(x) {}" +
"/** @type {(string,undefined)} */ var a;" +
"a && f(a);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: undefined");
}
public void testControlFlowRestrictsType7() throws Exception {
testTypes("/** @param {undefined} x */ function f(x) {}" +
"/** @type {(string,undefined)} */ var a;" +
"a && f(a);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: undefined");
}
public void testControlFlowRestrictsType8() throws Exception {
testTypes("/** @param {undefined} a */ function f(a){}" +
"/** @type {(!Array,undefined)} */ var a;" +
"if (a || f(a)) {}");
}
public void testControlFlowRestrictsType9() throws Exception {
testTypes("/** @param {number?} x\n * @return {number}*/\n" +
"var f = function(x) {\n" +
"if (!x || x == 1) { return 1; } else { return x; }\n" +
"};");
}
public void testControlFlowRestrictsType10() throws Exception {
// We should correctly infer that y will be (null|{}) because
// the loop wraps around.
testTypes("/** @param {number} x */ function f(x) {}" +
"function g() {" +
" var y = null;" +
" for (var i = 0; i < 10; i++) {" +
" f(y);" +
" if (y != null) {" +
" // y is None the first time it goes thru this branch\n" +
" } else {" +
" y = {};" +
" }" +
" }" +
"};",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (null|{})\n" +
"required: number");
}
public void testControlFlowRestrictsType11() throws Exception {
testTypes("/** @param {boolean} x */ function f(x) {}" +
"function g() {" +
" var y = null;" +
" if (y != null) {" +
" for (var i = 0; i < 10; i++) {" +
" f(y);" +
" }" +
" }" +
"};",
"condition always evaluates to false\n" +
"left : null\n" +
"right: null");
}
public void testSwitchCase3() throws Exception {
testTypes("/** @type String */" +
"var a = new String('foo');" +
"switch (a) { case 'A': }");
}
public void testSwitchCase4() throws Exception {
testTypes("/** @type {(string,Null)} */" +
"var a = 'foo';" +
"switch (a) { case 'A':break; case null:break; }");
}
public void testSwitchCase5() throws Exception {
testTypes("/** @type {(String,Null)} */" +
"var a = new String('foo');" +
"switch (a) { case 'A':break; case null:break; }");
}
public void testSwitchCase6() throws Exception {
testTypes("/** @type {(Number,Null)} */" +
"var a = new Number(5);" +
"switch (a) { case 5:break; case null:break; }");
}
public void testSwitchCase7() throws Exception {
// This really tests the inference inside the case.
testTypes(
"/**\n" +
" * @param {number} x\n" +
" * @return {number}\n" +
" */\n" +
"function g(x) { return 5; }" +
"function f() {" +
" var x = {};" +
" x.foo = '3';" +
" switch (3) { case g(x.foo): return 3; }" +
"}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testSwitchCase8() throws Exception {
// This really tests the inference inside the switch clause.
testTypes(
"/**\n" +
" * @param {number} x\n" +
" * @return {number}\n" +
" */\n" +
"function g(x) { return 5; }" +
"function f() {" +
" var x = {};" +
" x.foo = '3';" +
" switch (g(x.foo)) { case 3: return 3; }" +
"}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testNoTypeCheck1() throws Exception {
testTypes("/** @notypecheck */function foo() { new 4 }");
}
public void testNoTypeCheck2() throws Exception {
testTypes("/** @notypecheck */var foo = function() { new 4 }");
}
public void testNoTypeCheck3() throws Exception {
testTypes("/** @notypecheck */var foo = function bar() { new 4 }");
}
public void testNoTypeCheck4() throws Exception {
testTypes("var foo;" +
"/** @notypecheck */foo = function() { new 4 }");
}
public void testNoTypeCheck5() throws Exception {
testTypes("var foo;" +
"foo = /** @notypecheck */function() { new 4 }");
}
public void testNoTypeCheck6() throws Exception {
testTypes("var foo;" +
"/** @notypecheck */foo = function bar() { new 4 }");
}
public void testNoTypeCheck7() throws Exception {
testTypes("var foo;" +
"foo = /** @notypecheck */function bar() { new 4 }");
}
public void testNoTypeCheck8() throws Exception {
testTypes("/** @fileoverview \n * @notypecheck */ var foo;" +
"var bar = 3; /** @param {string} x */ function f(x) {} f(bar);");
}
public void testNoTypeCheck9() throws Exception {
testTypes("/** @notypecheck */ function g() { }" +
" /** @type {string} */ var a = 1",
"initializing variable\n" +
"found : number\n" +
"required: string"
);
}
public void testNoTypeCheck10() throws Exception {
testTypes("/** @notypecheck */ function g() { }" +
" function h() {/** @type {string} */ var a = 1}",
"initializing variable\n" +
"found : number\n" +
"required: string"
);
}
public void testNoTypeCheck11() throws Exception {
testTypes("/** @notypecheck */ function g() { }" +
"/** @notypecheck */ function h() {/** @type {string} */ var a = 1}"
);
}
public void testNoTypeCheck12() throws Exception {
testTypes("/** @notypecheck */ function g() { }" +
"function h() {/** @type {string}\n * @notypecheck\n*/ var a = 1}"
);
}
public void testNoTypeCheck13() throws Exception {
testTypes("/** @notypecheck */ function g() { }" +
"function h() {/** @type {string}\n * @notypecheck\n*/ var a = 1;" +
"/** @type {string}*/ var b = 1}",
"initializing variable\n" +
"found : number\n" +
"required: string"
);
}
public void testNoTypeCheck14() throws Exception {
testTypes("/** @fileoverview \n * @notypecheck */ function g() { }" +
"g(1,2,3)");
}
public void testImplicitCast() throws Exception {
testTypes("/** @constructor */ function Element() {};\n" +
"/** @type {string}\n" +
" * @implicitCast */" +
"Element.prototype.innerHTML;",
"(new Element).innerHTML = new Array();", null, false);
}
public void testImplicitCastSubclassAccess() throws Exception {
testTypes("/** @constructor */ function Element() {};\n" +
"/** @type {string}\n" +
" * @implicitCast */" +
"Element.prototype.innerHTML;" +
"/** @constructor \n @extends Element */" +
"function DIVElement() {};",
"(new DIVElement).innerHTML = new Array();",
null, false);
}
public void testImplicitCastNotInExterns() throws Exception {
testTypes("/** @constructor */ function Element() {};\n" +
"/** @type {string}\n" +
" * @implicitCast */" +
"Element.prototype.innerHTML;" +
"(new Element).innerHTML = new Array();",
new String[] {
"Illegal annotation on innerHTML. @implicitCast may only be " +
"used in externs.",
"assignment to property innerHTML of Element\n" +
"found : Array\n" +
"required: string"});
}
public void testNumberNode() throws Exception {
Node n = typeCheck(Node.newNumber(0));
assertEquals(NUMBER_TYPE, n.getJSType());
}
public void testStringNode() throws Exception {
Node n = typeCheck(Node.newString("hello"));
assertEquals(STRING_TYPE, n.getJSType());
}
public void testBooleanNodeTrue() throws Exception {
Node trueNode = typeCheck(new Node(Token.TRUE));
assertEquals(BOOLEAN_TYPE, trueNode.getJSType());
}
public void testBooleanNodeFalse() throws Exception {
Node falseNode = typeCheck(new Node(Token.FALSE));
assertEquals(BOOLEAN_TYPE, falseNode.getJSType());
}
public void testUndefinedNode() throws Exception {
Node p = new Node(Token.ADD);
Node n = Node.newString(Token.NAME, "undefined");
p.addChildToBack(n);
p.addChildToBack(Node.newNumber(5));
typeCheck(p);
assertEquals(VOID_TYPE, n.getJSType());
}
public void testNumberAutoboxing() throws Exception {
testTypes("/** @type Number */var a = 4;",
"initializing variable\n" +
"found : number\n" +
"required: (Number|null)");
}
public void testNumberUnboxing() throws Exception {
testTypes("/** @type number */var a = new Number(4);",
"initializing variable\n" +
"found : Number\n" +
"required: number");
}
public void testStringAutoboxing() throws Exception {
testTypes("/** @type String */var a = 'hello';",
"initializing variable\n" +
"found : string\n" +
"required: (String|null)");
}
public void testStringUnboxing() throws Exception {
testTypes("/** @type string */var a = new String('hello');",
"initializing variable\n" +
"found : String\n" +
"required: string");
}
public void testBooleanAutoboxing() throws Exception {
testTypes("/** @type Boolean */var a = true;",
"initializing variable\n" +
"found : boolean\n" +
"required: (Boolean|null)");
}
public void testBooleanUnboxing() throws Exception {
testTypes("/** @type boolean */var a = new Boolean(false);",
"initializing variable\n" +
"found : Boolean\n" +
"required: boolean");
}
public void testIssue86() throws Exception {
testTypes(
"/** @interface */ function I() {}" +
"/** @return {number} */ I.prototype.get = function(){};" +
"/** @constructor \n * @implements {I} */ function F() {}" +
"/** @override */ F.prototype.get = function() { return true; };",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testIssue124() throws Exception {
testTypes(
"var t = null;" +
"function test() {" +
" if (t != null) { t = null; }" +
" t = 1;" +
"}");
}
public void testIssue124b() throws Exception {
testTypes(
"var t = null;" +
"function test() {" +
" if (t != null) { t = null; }" +
" t = undefined;" +
"}",
"condition always evaluates to false\n" +
"left : (null|undefined)\n" +
"right: null");
}
public void testIssue259() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"/** @constructor */" +
"var Clock = function() {" +
" /** @constructor */" +
" this.Date = function() {};" +
" f(new this.Date());" +
"};",
"actual parameter 1 of f does not match formal parameter\n" +
"found : this.Date\n" +
"required: number");
}
public void testIssue301() throws Exception {
testTypes(
"Array.indexOf = function() {};" +
"var s = 'hello';" +
"alert(s.toLowerCase.indexOf('1'));",
"Property indexOf never defined on String.prototype.toLowerCase");
}
public void testIssue368() throws Exception {
testTypes(
"/** @constructor */ function Foo(){}" +
"/**\n" +
" * @param {number} one\n" +
" * @param {string} two\n" +
" */\n" +
"Foo.prototype.add = function(one, two) {};" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */\n" +
"function Bar(){}" +
"/** @override */\n" +
"Bar.prototype.add = function(ignored) {};" +
"(new Bar()).add(1, 2);",
"actual parameter 2 of Bar.prototype.add does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testIssue380() throws Exception {
testTypes(
"/** @type { function(string): {innerHTML: string} } */" +
"document.getElementById;" +
"var list = /** @type {!Array.<string>} */ ['hello', 'you'];\n" +
"list.push('?');\n" +
"document.getElementById('node').innerHTML = list.toString();");
}
public void testIssue483() throws Exception {
testTypes(
"/** @constructor */ function C() {" +
" /** @type {?Array} */ this.a = [];" +
"}" +
"C.prototype.f = function() {" +
" if (this.a.length > 0) {" +
" g(this.a);" +
" }" +
"};" +
"/** @param {number} a */ function g(a) {}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : Array\n" +
"required: number");
}
public void testIssue537a() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype = {method: function() {}};" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */\n" +
"function Bar() {" +
" Foo.call(this);" +
" if (this.baz()) this.method(1);" +
"}" +
"Bar.prototype = {" +
" baz: function() {" +
" return true;" +
" }" +
"};" +
"Bar.prototype.__proto__ = Foo.prototype;",
"Function Foo.prototype.method: called with 1 argument(s). " +
"Function requires at least 0 argument(s) " +
"and no more than 0 argument(s).");
}
public void testIssue537b() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype = {method: function() {}};" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */\n" +
"function Bar() {" +
" Foo.call(this);" +
" if (this.baz(1)) this.method();" +
"}" +
"Bar.prototype = {" +
" baz: function() {" +
" return true;" +
" }" +
"};" +
"Bar.prototype.__proto__ = Foo.prototype;",
"Function Bar.prototype.baz: called with 1 argument(s). " +
"Function requires at least 0 argument(s) " +
"and no more than 0 argument(s).");
}
public void testIssue537c() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */\n" +
"function Bar() {" +
" Foo.call(this);" +
" if (this.baz2()) alert(1);" +
"}" +
"Bar.prototype = {" +
" baz: function() {" +
" return true;" +
" }" +
"};" +
"Bar.prototype.__proto__ = Foo.prototype;",
"Property baz2 never defined on Bar");
}
public void testIssue537d() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype = {" +
" /** @return {Bar} */ x: function() { new Bar(); }," +
" /** @return {Foo} */ y: function() { new Bar(); }" +
"};" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */\n" +
"function Bar() {" +
" this.xy = 3;" +
"}" +
"/** @return {Bar} */ function f() { return new Bar(); }" +
"/** @return {Foo} */ function g() { return new Bar(); }" +
"Bar.prototype = {" +
" /** @return {Bar} */ x: function() { new Bar(); }," +
" /** @return {Foo} */ y: function() { new Bar(); }" +
"};" +
"Bar.prototype.__proto__ = Foo.prototype;");
}
public void testIssue586() throws Exception {
testTypes(
"/** @constructor */" +
"var MyClass = function() {};" +
"/** @param {boolean} success */" +
"MyClass.prototype.fn = function(success) {};" +
"MyClass.prototype.test = function() {" +
" this.fn();" +
" this.fn = function() {};" +
"};",
"Function MyClass.prototype.fn: called with 0 argument(s). " +
"Function requires at least 1 argument(s) " +
"and no more than 1 argument(s).");
}
public void testIssue635() throws Exception {
// TODO(nicksantos): Make this emit a warning, because of the 'this' type.
testTypes(
"/** @constructor */" +
"function F() {}" +
"F.prototype.bar = function() { this.baz(); };" +
"F.prototype.baz = function() {};" +
"/** @constructor */" +
"function G() {}" +
"G.prototype.bar = F.prototype.bar;");
}
public void testIssue669() throws Exception {
testTypes(
"/** @return {{prop1: (Object|undefined)}} */" +
"function f(a) {" +
" var results;" +
" if (a) {" +
" results = {};" +
" results.prop1 = {a: 3};" +
" } else {" +
" results = {prop2: 3};" +
" }" +
" return results;" +
"}");
}
public void testIssue700() throws Exception {
testTypes(
"/**\n" +
" * @param {{text: string}} opt_data\n" +
" * @return {string}\n" +
" */\n" +
"function temp1(opt_data) {\n" +
" return opt_data.text;\n" +
"}\n" +
"\n" +
"/**\n" +
" * @param {{activity: (boolean|number|string|null|Object)}} opt_data\n" +
" * @return {string}\n" +
" */\n" +
"function temp2(opt_data) {\n" +
" /** @notypecheck */\n" +
" function __inner() {\n" +
" return temp1(opt_data.activity);\n" +
" }\n" +
" return __inner();\n" +
"}\n" +
"\n" +
"/**\n" +
" * @param {{n: number, text: string, b: boolean}} opt_data\n" +
" * @return {string}\n" +
" */\n" +
"function temp3(opt_data) {\n" +
" return 'n: ' + opt_data.n + ', t: ' + opt_data.text + '.';\n" +
"}\n" +
"\n" +
"function callee() {\n" +
" var output = temp3({\n" +
" n: 0,\n" +
" text: 'a string',\n" +
" b: true\n" +
" })\n" +
" alert(output);\n" +
"}\n" +
"\n" +
"callee();");
}
/**
* Tests that the || operator is type checked correctly, that is of
* the type of the first argument or of the second argument. See
* bugid 592170 for more details.
*/
public void testBug592170() throws Exception {
testTypes(
"/** @param {Function} opt_f ... */" +
"function foo(opt_f) {" +
" /** @type {Function} */" +
" return opt_f || function () {};" +
"}");
}
/**
* Tests that undefined can be compared shallowly to a value of type
* (number,undefined) regardless of the side on which the undefined
* value is.
*/
public void testBug901455() throws Exception {
testTypes("/** @return {(number,undefined)} */ function a() { return 3; }" +
"var b = undefined === a()");
testTypes("/** @return {(number,undefined)} */ function a() { return 3; }" +
"var b = a() === undefined");
}
/**
* Tests that the match method of strings returns nullable arrays.
*/
public void testBug908701() throws Exception {
testTypes("/** @type {String} */var s = new String('foo');" +
"var b = s.match(/a/) != null;");
}
/**
* Tests that named types play nicely with subtyping.
*/
public void testBug908625() throws Exception {
testTypes("/** @constructor */function A(){}" +
"/** @constructor\n * @extends A */function B(){}" +
"/** @param {B} b" +
"\n @return {(A,undefined)} */function foo(b){return b}");
}
/**
* Tests that assigning two untyped functions to a variable whose type is
* inferred and calling this variable is legal.
*/
public void testBug911118() throws Exception {
// verifying the type assigned to function expressions assigned variables
Scope s = parseAndTypeCheckWithScope("var a = function(){};").scope;
JSType type = s.getVar("a").getType();
assertEquals("function (): undefined", type.toString());
// verifying the bug example
testTypes("function nullFunction() {};" +
"var foo = nullFunction;" +
"foo = function() {};" +
"foo();");
}
public void testBug909000() throws Exception {
testTypes("/** @constructor */function A(){}\n" +
"/** @param {!A} a\n" +
"@return {boolean}*/\n" +
"function y(a) { return a }",
"inconsistent return type\n" +
"found : A\n" +
"required: boolean");
}
public void testBug930117() throws Exception {
testTypes(
"/** @param {boolean} x */function f(x){}" +
"f(null);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : null\n" +
"required: boolean");
}
public void testBug1484445() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @type {number?} */ Foo.prototype.bar = null;" +
"/** @type {number?} */ Foo.prototype.baz = null;" +
"/** @param {Foo} foo */" +
"function f(foo) {" +
" while (true) {" +
" if (foo.bar == null && foo.baz == null) {" +
" foo.bar;" +
" }" +
" }" +
"}");
}
public void testBug1859535() throws Exception {
testTypes(
"/**\n" +
" * @param {Function} childCtor Child class.\n" +
" * @param {Function} parentCtor Parent class.\n" +
" */" +
"var inherits = function(childCtor, parentCtor) {" +
" /** @constructor */" +
" function tempCtor() {};" +
" tempCtor.prototype = parentCtor.prototype;" +
" childCtor.superClass_ = parentCtor.prototype;" +
" childCtor.prototype = new tempCtor();" +
" /** @override */ childCtor.prototype.constructor = childCtor;" +
"};" +
"/**" +
" * @param {Function} constructor\n" +
" * @param {Object} var_args\n" +
" * @return {Object}\n" +
" */" +
"var factory = function(constructor, var_args) {" +
" /** @constructor */" +
" var tempCtor = function() {};" +
" tempCtor.prototype = constructor.prototype;" +
" var obj = new tempCtor();" +
" constructor.apply(obj, arguments);" +
" return obj;" +
"};");
}
public void testBug1940591() throws Exception {
testTypes(
"/** @type {Object} */" +
"var a = {};\n" +
"/** @type {number} */\n" +
"a.name = 0;\n" +
"/**\n" +
" * @param {Function} x anything.\n" +
" */\n" +
"a.g = function(x) { x.name = 'a'; }");
}
public void testBug1942972() throws Exception {
testTypes(
"var google = {\n" +
" gears: {\n" +
" factory: {},\n" +
" workerPool: {}\n" +
" }\n" +
"};\n" +
"\n" +
"google.gears = {factory: {}};\n");
}
public void testBug1943776() throws Exception {
testTypes(
"/** @return {{foo: Array}} */" +
"function bar() {" +
" return {foo: []};" +
"}");
}
public void testBug1987544() throws Exception {
testTypes(
"/** @param {string} x */ function foo(x) {}" +
"var duration;" +
"if (true && !(duration = 3)) {" +
" foo(duration);" +
"}",
"actual parameter 1 of foo does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testBug1940769() throws Exception {
testTypes(
"/** @return {!Object} */ " +
"function proto(obj) { return obj.prototype; }" +
"/** @constructor */ function Map() {}" +
"/**\n" +
" * @constructor\n" +
" * @extends {Map}\n" +
" */" +
"function Map2() { Map.call(this); };" +
"Map2.prototype = proto(Map);");
}
public void testBug2335992() throws Exception {
testTypes(
"/** @return {*} */ function f() { return 3; }" +
"var x = f();" +
"/** @type {string} */" +
"x.y = 3;",
"assignment\n" +
"found : number\n" +
"required: string");
}
public void testBug2341812() throws Exception {
testTypes(
"/** @interface */" +
"function EventTarget() {}" +
"/** @constructor \n * @implements {EventTarget} */" +
"function Node() {}" +
"/** @type {number} */ Node.prototype.index;" +
"/** @param {EventTarget} x \n * @return {string} */" +
"function foo(x) { return x.index; }");
}
public void testScopedConstructors1() throws Exception {
testTypes(
"function foo1() { " +
" /** @constructor */ function Bar() { " +
" /** @type {number} */ this.x = 3;" +
" }" +
"}" +
"function foo2() { " +
" /** @constructor */ function Bar() { " +
" /** @type {string} */ this.x = 'y';" +
" }" +
" /** " +
" * @param {Bar} b\n" +
" * @return {number}\n" +
" */" +
" function baz(b) { return b.x; }" +
"}",
"inconsistent return type\n" +
"found : string\n" +
"required: number");
}
public void testScopedConstructors2() throws Exception {
testTypes(
"/** @param {Function} f */" +
"function foo1(f) {" +
" /** @param {Function} g */" +
" f.prototype.bar = function(g) {};" +
"}");
}
public void testQualifiedNameInference1() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @type {number?} */ Foo.prototype.bar = null;" +
"/** @type {number?} */ Foo.prototype.baz = null;" +
"/** @param {Foo} foo */" +
"function f(foo) {" +
" while (true) {" +
" if (!foo.baz) break; " +
" foo.bar = null;" +
" }" +
// Tests a bug where this condition always evaluated to true.
" return foo.bar == null;" +
"}");
}
public void testQualifiedNameInference2() throws Exception {
testTypes(
"var x = {};" +
"x.y = c;" +
"function f(a, b) {" +
" if (a) {" +
" if (b) " +
" x.y = 2;" +
" else " +
" x.y = 1;" +
" }" +
" return x.y == null;" +
"}");
}
public void testQualifiedNameInference3() throws Exception {
testTypes(
"var x = {};" +
"x.y = c;" +
"function f(a, b) {" +
" if (a) {" +
" if (b) " +
" x.y = 2;" +
" else " +
" x.y = 1;" +
" }" +
" return x.y == null;" +
"} function g() { x.y = null; }");
}
public void testQualifiedNameInference4() throws Exception {
testTypes(
"/** @param {string} x */ function f(x) {}\n" +
"/**\n" +
" * @param {?string} x \n" +
" * @constructor\n" +
" */" +
"function Foo(x) { this.x_ = x; }\n" +
"Foo.prototype.bar = function() {" +
" if (this.x_) { f(this.x_); }" +
"};");
}
public void testQualifiedNameInference5() throws Exception {
testTypes(
"var ns = {}; " +
"(function() { " +
" /** @param {number} x */ ns.foo = function(x) {}; })();" +
"(function() { ns.foo(true); })();",
"actual parameter 1 of ns.foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testQualifiedNameInference6() throws Exception {
testTypes(
"var ns = {}; " +
"/** @param {number} x */ ns.foo = function(x) {};" +
"(function() { " +
" ns.foo = function(x) {};" +
" ns.foo(true); })();",
"actual parameter 1 of ns.foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testQualifiedNameInference7() throws Exception {
testTypes(
"var ns = {}; " +
"(function() { " +
" /** @constructor \n * @param {number} x */ " +
" ns.Foo = function(x) {};" +
" /** @param {ns.Foo} x */ function f(x) {}" +
" f(new ns.Foo(true));" +
"})();",
"actual parameter 1 of ns.Foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testQualifiedNameInference8() throws Exception {
testTypes(
"var ns = {}; " +
"(function() { " +
" /** @constructor \n * @param {number} x */ " +
" ns.Foo = function(x) {};" +
"})();" +
"/** @param {ns.Foo} x */ function f(x) {}" +
"f(new ns.Foo(true));",
"Bad type annotation. Unknown type ns.Foo");
}
public void testQualifiedNameInference9() throws Exception {
testTypes(
"var ns = {}; " +
"ns.ns2 = {}; " +
"(function() { " +
" /** @constructor \n * @param {number} x */ " +
" ns.ns2.Foo = function(x) {};" +
" /** @param {ns.ns2.Foo} x */ function f(x) {}" +
" f(new ns.ns2.Foo(true));" +
"})();",
"actual parameter 1 of ns.ns2.Foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testQualifiedNameInference10() throws Exception {
testTypes(
"var ns = {}; " +
"ns.ns2 = {}; " +
"(function() { " +
" /** @interface */ " +
" ns.ns2.Foo = function() {};" +
" /** @constructor \n * @implements {ns.ns2.Foo} */ " +
" function F() {}" +
" (new F());" +
"})();");
}
public void testQualifiedNameInference11() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"function f() {" +
" var x = new Foo();" +
" x.onload = function() {" +
" x.onload = null;" +
" };" +
"}");
}
public void testQualifiedNameInference12() throws Exception {
// We should be able to tell that the two 'this' properties
// are different.
testTypes(
"/** @param {function(this:Object)} x */ function f(x) {}" +
"/** @constructor */ function Foo() {" +
" /** @type {number} */ this.bar = 3;" +
" f(function() { this.bar = true; });" +
"}");
}
public void testSheqRefinedScope() throws Exception {
Node n = parseAndTypeCheck(
"/** @constructor */function A() {}\n" +
"/** @constructor \n @extends A */ function B() {}\n" +
"/** @return {number} */\n" +
"B.prototype.p = function() { return 1; }\n" +
"/** @param {A} a\n @param {B} b */\n" +
"function f(a, b) {\n" +
" b.p();\n" +
" if (a === b) {\n" +
" b.p();\n" +
" }\n" +
"}");
Node nodeC = n.getLastChild().getLastChild().getLastChild().getLastChild()
.getLastChild().getLastChild();
JSType typeC = nodeC.getJSType();
assertTrue(typeC.isNumber());
Node nodeB = nodeC.getFirstChild().getFirstChild();
JSType typeB = nodeB.getJSType();
assertEquals("B", typeB.toString());
}
public void testAssignToUntypedVariable() throws Exception {
Node n = parseAndTypeCheck("var z; z = 1;");
Node assign = n.getLastChild().getFirstChild();
Node node = assign.getFirstChild();
assertFalse(node.getJSType().isUnknownType());
assertEquals("number", node.getJSType().toString());
}
public void testAssignToUntypedProperty() throws Exception {
Node n = parseAndTypeCheck(
"/** @constructor */ function Foo() {}\n" +
"Foo.prototype.a = 1;" +
"(new Foo).a;");
Node node = n.getLastChild().getFirstChild();
assertFalse(node.getJSType().isUnknownType());
assertTrue(node.getJSType().isNumber());
}
public void testNew1() throws Exception {
testTypes("new 4", TypeCheck.NOT_A_CONSTRUCTOR);
}
public void testNew2() throws Exception {
testTypes("var Math = {}; new Math()", TypeCheck.NOT_A_CONSTRUCTOR);
}
public void testNew3() throws Exception {
testTypes("new Date()");
}
public void testNew4() throws Exception {
testTypes("/** @constructor */function A(){}; new A();");
}
public void testNew5() throws Exception {
testTypes("function A(){}; new A();", TypeCheck.NOT_A_CONSTRUCTOR);
}
public void testNew6() throws Exception {
TypeCheckResult p =
parseAndTypeCheckWithScope("/** @constructor */function A(){};" +
"var a = new A();");
JSType aType = p.scope.getVar("a").getType();
assertTrue(aType instanceof ObjectType);
ObjectType aObjectType = (ObjectType) aType;
assertEquals("A", aObjectType.getConstructor().getReferenceName());
}
public void testNew7() throws Exception {
testTypes("/** @param {Function} opt_constructor */" +
"function foo(opt_constructor) {" +
"if (opt_constructor) { new opt_constructor; }" +
"}");
}
public void testNew8() throws Exception {
testTypes("/** @param {Function} opt_constructor */" +
"function foo(opt_constructor) {" +
"new opt_constructor;" +
"}");
}
public void testNew9() throws Exception {
testTypes("/** @param {Function} opt_constructor */" +
"function foo(opt_constructor) {" +
"new (opt_constructor || Array);" +
"}");
}
public void testNew10() throws Exception {
testTypes("var goog = {};" +
"/** @param {Function} opt_constructor */" +
"goog.Foo = function (opt_constructor) {" +
"new (opt_constructor || Array);" +
"}");
}
public void testNew11() throws Exception {
testTypes("/** @param {Function} c1 */" +
"function f(c1) {" +
" var c2 = function(){};" +
" c1.prototype = new c2;" +
"}", TypeCheck.NOT_A_CONSTRUCTOR);
}
public void testNew12() throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope("var a = new Array();");
Var a = p.scope.getVar("a");
assertEquals(ARRAY_TYPE, a.getType());
}
public void testNew13() throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope(
"/** @constructor */function FooBar(){};" +
"var a = new FooBar();");
Var a = p.scope.getVar("a");
assertTrue(a.getType() instanceof ObjectType);
assertEquals("FooBar", a.getType().toString());
}
public void testNew14() throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope(
"/** @constructor */var FooBar = function(){};" +
"var a = new FooBar();");
Var a = p.scope.getVar("a");
assertTrue(a.getType() instanceof ObjectType);
assertEquals("FooBar", a.getType().toString());
}
public void testNew15() throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope(
"var goog = {};" +
"/** @constructor */goog.A = function(){};" +
"var a = new goog.A();");
Var a = p.scope.getVar("a");
assertTrue(a.getType() instanceof ObjectType);
assertEquals("goog.A", a.getType().toString());
}
public void testNew16() throws Exception {
testTypes(
"/** \n" +
" * @param {string} x \n" +
" * @constructor \n" +
" */" +
"function Foo(x) {}" +
"function g() { new Foo(1); }",
"actual parameter 1 of Foo does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testNew17() throws Exception {
testTypes("var goog = {}; goog.x = 3; new goog.x",
"cannot instantiate non-constructor");
}
public void testNew18() throws Exception {
testTypes("var goog = {};" +
"/** @constructor */ goog.F = function() {};" +
"/** @constructor */ goog.G = goog.F;");
}
public void testName1() throws Exception {
assertEquals(VOID_TYPE, testNameNode("undefined"));
}
public void testName2() throws Exception {
assertEquals(OBJECT_FUNCTION_TYPE, testNameNode("Object"));
}
public void testName3() throws Exception {
assertEquals(ARRAY_FUNCTION_TYPE, testNameNode("Array"));
}
public void testName4() throws Exception {
assertEquals(DATE_FUNCTION_TYPE, testNameNode("Date"));
}
public void testName5() throws Exception {
assertEquals(REGEXP_FUNCTION_TYPE, testNameNode("RegExp"));
}
/**
* Type checks a NAME node and retrieve its type.
*/
private JSType testNameNode(String name) {
Node node = Node.newString(Token.NAME, name);
Node parent = new Node(Token.SCRIPT, node);
parent.setInputId(new InputId("code"));
Node externs = new Node(Token.SCRIPT);
externs.setInputId(new InputId("externs"));
Node externAndJsRoot = new Node(Token.BLOCK, externs, parent);
externAndJsRoot.setIsSyntheticBlock(true);
makeTypeCheck().processForTesting(null, parent);
return node.getJSType();
}
public void testBitOperation1() throws Exception {
testTypes("/**@return {void}*/function foo(){ ~foo(); }",
"operator ~ cannot be applied to undefined");
}
public void testBitOperation2() throws Exception {
testTypes("/**@return {void}*/function foo(){var a = foo()<<3;}",
"operator << cannot be applied to undefined");
}
public void testBitOperation3() throws Exception {
testTypes("/**@return {void}*/function foo(){var a = 3<<foo();}",
"operator << cannot be applied to undefined");
}
public void testBitOperation4() throws Exception {
testTypes("/**@return {void}*/function foo(){var a = foo()>>>3;}",
"operator >>> cannot be applied to undefined");
}
public void testBitOperation5() throws Exception {
testTypes("/**@return {void}*/function foo(){var a = 3>>>foo();}",
"operator >>> cannot be applied to undefined");
}
public void testBitOperation6() throws Exception {
testTypes("/**@return {!Object}*/function foo(){var a = foo()&3;}",
"bad left operand to bitwise operator\n" +
"found : Object\n" +
"required: (boolean|null|number|string|undefined)");
}
public void testBitOperation7() throws Exception {
testTypes("var x = null; x |= undefined; x &= 3; x ^= '3'; x |= true;");
}
public void testBitOperation8() throws Exception {
testTypes("var x = void 0; x |= new Number(3);");
}
public void testBitOperation9() throws Exception {
testTypes("var x = void 0; x |= {};",
"bad right operand to bitwise operator\n" +
"found : {}\n" +
"required: (boolean|null|number|string|undefined)");
}
public void testCall1() throws Exception {
testTypes("3();", "number expressions are not callable");
}
public void testCall2() throws Exception {
testTypes("/** @param {!Number} foo*/function bar(foo){ bar('abc'); }",
"actual parameter 1 of bar does not match formal parameter\n" +
"found : string\n" +
"required: Number");
}
public void testCall3() throws Exception {
// We are checking that an unresolved named type can successfully
// meet with a functional type to produce a callable type.
testTypes("/** @type {Function|undefined} */var opt_f;" +
"/** @type {some.unknown.type} */var f1;" +
"var f2 = opt_f || f1;" +
"f2();",
"Bad type annotation. Unknown type some.unknown.type");
}
public void testCall4() throws Exception {
testTypes("/**@param {!RegExp} a*/var foo = function bar(a){ bar('abc'); }",
"actual parameter 1 of bar does not match formal parameter\n" +
"found : string\n" +
"required: RegExp");
}
public void testCall5() throws Exception {
testTypes("/**@param {!RegExp} a*/var foo = function bar(a){ foo('abc'); }",
"actual parameter 1 of foo does not match formal parameter\n" +
"found : string\n" +
"required: RegExp");
}
public void testCall6() throws Exception {
testTypes("/** @param {!Number} foo*/function bar(foo){}" +
"bar('abc');",
"actual parameter 1 of bar does not match formal parameter\n" +
"found : string\n" +
"required: Number");
}
public void testCall7() throws Exception {
testTypes("/** @param {!RegExp} a*/var foo = function bar(a){};" +
"foo('abc');",
"actual parameter 1 of foo does not match formal parameter\n" +
"found : string\n" +
"required: RegExp");
}
public void testCall8() throws Exception {
testTypes("/** @type {Function|number} */var f;f();",
"(Function|number) expressions are " +
"not callable");
}
public void testCall9() throws Exception {
testTypes(
"var goog = {};" +
"/** @constructor */ goog.Foo = function() {};" +
"/** @param {!goog.Foo} a */ var bar = function(a){};" +
"bar('abc');",
"actual parameter 1 of bar does not match formal parameter\n" +
"found : string\n" +
"required: goog.Foo");
}
public void testCall10() throws Exception {
testTypes("/** @type {Function} */var f;f();");
}
public void testCall11() throws Exception {
testTypes("var f = new Function(); f();");
}
public void testFunctionCall1() throws Exception {
testTypes(
"/** @param {number} x */ var foo = function(x) {};" +
"foo.call(null, 3);");
}
public void testFunctionCall2() throws Exception {
testTypes(
"/** @param {number} x */ var foo = function(x) {};" +
"foo.call(null, 'bar');",
"actual parameter 2 of foo.call does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testFunctionCall3() throws Exception {
testTypes(
"/** @param {number} x \n * @constructor */ " +
"var Foo = function(x) { this.bar.call(null, x); };" +
"/** @type {function(number)} */ Foo.prototype.bar;");
}
public void testFunctionCall4() throws Exception {
testTypes(
"/** @param {string} x \n * @constructor */ " +
"var Foo = function(x) { this.bar.call(null, x); };" +
"/** @type {function(number)} */ Foo.prototype.bar;",
"actual parameter 2 of this.bar.call " +
"does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testFunctionCall5() throws Exception {
testTypes(
"/** @param {Function} handler \n * @constructor */ " +
"var Foo = function(handler) { handler.call(this, x); };");
}
public void testFunctionCall6() throws Exception {
testTypes(
"/** @param {Function} handler \n * @constructor */ " +
"var Foo = function(handler) { handler.apply(this, x); };");
}
public void testFunctionCall7() throws Exception {
testTypes(
"/** @param {Function} handler \n * @param {Object} opt_context */ " +
"var Foo = function(handler, opt_context) { " +
" handler.call(opt_context, x);" +
"};");
}
public void testFunctionCall8() throws Exception {
testTypes(
"/** @param {Function} handler \n * @param {Object} opt_context */ " +
"var Foo = function(handler, opt_context) { " +
" handler.apply(opt_context, x);" +
"};");
}
public void testFunctionBind1() throws Exception {
testTypes(
"/** @type {function(string, number): boolean} */" +
"function f(x, y) { return true; }" +
"f.bind(null, 3);",
"actual parameter 2 of f.bind does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testFunctionBind2() throws Exception {
testTypes(
"/** @type {function(number): boolean} */" +
"function f(x) { return true; }" +
"f(f.bind(null, 3)());",
"actual parameter 1 of f does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testFunctionBind3() throws Exception {
testTypes(
"/** @type {function(number, string): boolean} */" +
"function f(x, y) { return true; }" +
"f.bind(null, 3)(true);",
"actual parameter 1 of function does not match formal parameter\n" +
"found : boolean\n" +
"required: string");
}
public void testFunctionBind4() throws Exception {
testTypes(
"/** @param {...number} x */" +
"function f(x) {}" +
"f.bind(null, 3, 3, 3)(true);",
"actual parameter 1 of function does not match formal parameter\n" +
"found : boolean\n" +
"required: (number|undefined)");
}
public void testFunctionBind5() throws Exception {
testTypes(
"/** @param {...number} x */" +
"function f(x) {}" +
"f.bind(null, true)(3, 3, 3);",
"actual parameter 2 of f.bind does not match formal parameter\n" +
"found : boolean\n" +
"required: (number|undefined)");
}
public void testGoogBind1() throws Exception {
// We currently do not support goog.bind natively.
testClosureTypes(
"var goog = {}; goog.bind = function(var_args) {};" +
"/** @type {function(number): boolean} */" +
"function f(x, y) { return true; }" +
"f(goog.bind(f, null, 'x')());",
null);
}
public void testCast2() throws Exception {
// can upcast to a base type.
testTypes("/** @constructor */function base() {}\n" +
"/** @constructor\n @extends {base} */function derived() {}\n" +
"/** @type {base} */ var baz = new derived();\n");
}
public void testCast3() throws Exception {
// cannot downcast
testTypes("/** @constructor */function base() {}\n" +
"/** @constructor @extends {base} */function derived() {}\n" +
"/** @type {!derived} */ var baz = new base();\n",
"initializing variable\n" +
"found : base\n" +
"required: derived");
}
public void testCast4() throws Exception {
// downcast must be explicit
testTypes("/** @constructor */function base() {}\n" +
"/** @constructor\n * @extends {base} */function derived() {}\n" +
"/** @type {!derived} */ var baz = " +
"/** @type {!derived} */(new base());\n");
}
public void testCast5() throws Exception {
// cannot explicitly cast to an unrelated type
testTypes("/** @constructor */function foo() {}\n" +
"/** @constructor */function bar() {}\n" +
"var baz = /** @type {!foo} */(new bar);\n",
"invalid cast - must be a subtype or supertype\n" +
"from: bar\n" +
"to : foo");
}
public void testCast6() throws Exception {
// can explicitly cast to a subtype or supertype
testTypes("/** @constructor */function foo() {}\n" +
"/** @constructor \n @extends foo */function bar() {}\n" +
"var baz = /** @type {!bar} */(new bar);\n" +
"var baz = /** @type {!foo} */(new foo);\n" +
"var baz = /** @type {bar} */(new bar);\n" +
"var baz = /** @type {foo} */(new foo);\n" +
"var baz = /** @type {!foo} */(new bar);\n" +
"var baz = /** @type {!bar} */(new foo);\n" +
"var baz = /** @type {foo} */(new bar);\n" +
"var baz = /** @type {bar} */(new foo);\n");
}
public void testCast7() throws Exception {
testTypes("var x = /** @type {foo} */ (new Object());",
"Bad type annotation. Unknown type foo");
}
public void testCast8() throws Exception {
testTypes("function f() { return /** @type {foo} */ (new Object()); }",
"Bad type annotation. Unknown type foo");
}
public void testCast9() throws Exception {
testTypes("var foo = {};" +
"function f() { return /** @type {foo} */ (new Object()); }",
"Bad type annotation. Unknown type foo");
}
public void testCast10() throws Exception {
testTypes("var foo = function() {};" +
"function f() { return /** @type {foo} */ (new Object()); }",
"Bad type annotation. Unknown type foo");
}
public void testCast11() throws Exception {
testTypes("var goog = {}; goog.foo = {};" +
"function f() { return /** @type {goog.foo} */ (new Object()); }",
"Bad type annotation. Unknown type goog.foo");
}
public void testCast12() throws Exception {
testTypes("var goog = {}; goog.foo = function() {};" +
"function f() { return /** @type {goog.foo} */ (new Object()); }",
"Bad type annotation. Unknown type goog.foo");
}
public void testCast13() throws Exception {
// Test to make sure that the forward-declaration still allows for
// a warning.
testClosureTypes("var goog = {}; " +
"goog.addDependency('zzz.js', ['goog.foo'], []);" +
"goog.foo = function() {};" +
"function f() { return /** @type {goog.foo} */ (new Object()); }",
"Bad type annotation. Unknown type goog.foo");
}
public void testCast14() throws Exception {
// Test to make sure that the forward-declaration still prevents
// some warnings.
testClosureTypes("var goog = {}; " +
"goog.addDependency('zzz.js', ['goog.bar'], []);" +
"function f() { return /** @type {goog.bar} */ (new Object()); }",
null);
}
public void testCast15() throws Exception {
// This fixes a bug where a type cast on an object literal
// would cause a runtime cast exception if the node was visited
// more than once.
//
// Some code assumes that an object literal must have a object type,
// while because of the cast, it could have any type (including
// a union).
testTypes(
"for (var i = 0; i < 10; i++) {" +
"var x = /** @type {Object|number} */ ({foo: 3});" +
"/** @param {number} x */ function f(x) {}" +
"f(x.foo);" +
"f([].foo);" +
"}",
"Property foo never defined on Array");
}
public void testCast16() throws Exception {
// A type cast should not invalidate the checks on the members
testTypes(
"for (var i = 0; i < 10; i++) {" +
"var x = /** @type {Object|number} */ (" +
" {/** @type {string} */ foo: 3});" +
"}",
"assignment to property foo of Object\n" +
"found : number\n" +
"required: string");
}
public void testCast17() throws Exception {
// Mostly verifying that rhino actually understands these JsDocs.
testTypes("/** @constructor */ function Foo() {} \n" +
"/** @type {Foo} */ var x = /** @type {Foo} */ ({})");
testTypes("/** @constructor */ function Foo() {} \n" +
"/** @type {Foo} */ var x = (/** @type {Foo} */ {})");
// Not really encourage because of possible ambiguity but it works.
testTypes("/** @constructor */ function Foo() {} \n" +
"/** @type {Foo} */ var x = /** @type {Foo} */ {}");
}
public void testNestedCasts() throws Exception {
testTypes("/** @constructor */var T = function() {};\n" +
"/** @constructor */var V = function() {};\n" +
"/**\n" +
"* @param {boolean} b\n" +
"* @return {T|V}\n" +
"*/\n" +
"function f(b) { return b ? new T() : new V(); }\n" +
"/**\n" +
"* @param {boolean} b\n" +
"* @return {boolean|undefined}\n" +
"*/\n" +
"function g(b) { return b ? true : undefined; }\n" +
"/** @return {T} */\n" +
"function h() {\n" +
"return /** @type {T} */ (f(/** @type {boolean} */ (g(true))));\n" +
"}");
}
public void testNativeCast1() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"f(String(true));",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testNativeCast2() throws Exception {
testTypes(
"/** @param {string} x */ function f(x) {}" +
"f(Number(true));",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testNativeCast3() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"f(Boolean(''));",
"actual parameter 1 of f does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testNativeCast4() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"f(Error(''));",
"actual parameter 1 of f does not match formal parameter\n" +
"found : Error\n" +
"required: number");
}
public void testBadConstructorCall() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo();",
"Constructor function (new:Foo): undefined should be called " +
"with the \"new\" keyword");
}
public void testTypeof() throws Exception {
testTypes("/**@return {void}*/function foo(){ var a = typeof foo(); }");
}
public void testConstructorType1() throws Exception {
testTypes("/**@constructor*/function Foo(){}" +
"/**@type{!Foo}*/var f = new Date();",
"initializing variable\n" +
"found : Date\n" +
"required: Foo");
}
public void testConstructorType2() throws Exception {
testTypes("/**@constructor*/function Foo(){\n" +
"/**@type{Number}*/this.bar = new Number(5);\n" +
"}\n" +
"/**@type{Foo}*/var f = new Foo();\n" +
"/**@type{Number}*/var n = f.bar;");
}
public void testConstructorType3() throws Exception {
// Reverse the declaration order so that we know that Foo is getting set
// even on an out-of-order declaration sequence.
testTypes("/**@type{Foo}*/var f = new Foo();\n" +
"/**@type{Number}*/var n = f.bar;" +
"/**@constructor*/function Foo(){\n" +
"/**@type{Number}*/this.bar = new Number(5);\n" +
"}\n");
}
public void testConstructorType4() throws Exception {
testTypes("/**@constructor*/function Foo(){\n" +
"/**@type{!Number}*/this.bar = new Number(5);\n" +
"}\n" +
"/**@type{!Foo}*/var f = new Foo();\n" +
"/**@type{!String}*/var n = f.bar;",
"initializing variable\n" +
"found : Number\n" +
"required: String");
}
public void testConstructorType5() throws Exception {
testTypes("/**@constructor*/function Foo(){}\n" +
"if (Foo){}\n");
}
public void testConstructorType6() throws Exception {
testTypes("/** @constructor */\n" +
"function bar() {}\n" +
"function _foo() {\n" +
" /** @param {bar} x */\n" +
" function f(x) {}\n" +
"}");
}
public void testConstructorType7() throws Exception {
TypeCheckResult p =
parseAndTypeCheckWithScope("/** @constructor */function A(){};");
JSType type = p.scope.getVar("A").getType();
assertTrue(type instanceof FunctionType);
FunctionType fType = (FunctionType) type;
assertEquals("A", fType.getReferenceName());
}
public void testConstructorType8() throws Exception {
testTypes(
"var ns = {};" +
"ns.create = function() { return function() {}; };" +
"/** @constructor */ ns.Foo = ns.create();" +
"ns.Foo.prototype = {x: 0, y: 0};" +
"/**\n" +
" * @param {ns.Foo} foo\n" +
" * @return {string}\n" +
" */\n" +
"function f(foo) {" +
" return foo.x;" +
"}",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testConstructorType9() throws Exception {
testTypes(
"var ns = {};" +
"ns.create = function() { return function() {}; };" +
"ns.extend = function(x) { return x; };" +
"/** @constructor */ ns.Foo = ns.create();" +
"ns.Foo.prototype = ns.extend({x: 0, y: 0});" +
"/**\n" +
" * @param {ns.Foo} foo\n" +
" * @return {string}\n" +
" */\n" +
"function f(foo) {" +
" return foo.x;" +
"}");
}
public void testAnonymousPrototype1() throws Exception {
testTypes(
"var ns = {};" +
"/** @constructor */ ns.Foo = function() {" +
" this.bar(3, 5);" +
"};" +
"ns.Foo.prototype = {" +
" bar: function(x) {}" +
"};",
"Function ns.Foo.prototype.bar: called with 2 argument(s). " +
"Function requires at least 1 argument(s) and no more " +
"than 1 argument(s).");
}
public void testAnonymousPrototype2() throws Exception {
testTypes(
"/** @interface */ var Foo = function() {};" +
"Foo.prototype = {" +
" foo: function(x) {}" +
"};" +
"/**\n" +
" * @constructor\n" +
" * @implements {Foo}\n" +
" */ var Bar = function() {};",
"property foo on interface Foo is not implemented by type Bar");
}
public void testAnonymousType1() throws Exception {
testTypes("function f() {}" +
"/** @constructor */\n" +
"f().bar = function() {};");
}
public void testAnonymousType2() throws Exception {
testTypes("function f() {}" +
"/** @interface */\n" +
"f().bar = function() {};");
}
public void testAnonymousType3() throws Exception {
testTypes("function f() {}" +
"/** @enum */\n" +
"f().bar = {FOO: 1};");
}
public void testBang1() throws Exception {
testTypes("/** @param {Object} x\n@return {!Object} */\n" +
"function f(x) { return x; }",
"inconsistent return type\n" +
"found : (Object|null)\n" +
"required: Object");
}
public void testBang2() throws Exception {
testTypes("/** @param {Object} x\n@return {!Object} */\n" +
"function f(x) { return x ? x : new Object(); }");
}
public void testBang3() throws Exception {
testTypes("/** @param {Object} x\n@return {!Object} */\n" +
"function f(x) { return /** @type {!Object} */ (x); }");
}
public void testBang4() throws Exception {
testTypes("/**@param {Object} x\n@param {Object} y\n@return {boolean}*/\n" +
"function f(x, y) {\n" +
"if (typeof x != 'undefined') { return x == y; }\n" +
"else { return x != y; }\n}");
}
public void testBang5() throws Exception {
testTypes("/**@param {Object} x\n@param {Object} y\n@return {boolean}*/\n" +
"function f(x, y) { return !!x && x == y; }");
}
public void testBang6() throws Exception {
testTypes("/** @param {Object?} x\n@return {Object} */\n" +
"function f(x) { return x; }");
}
public void testBang7() throws Exception {
testTypes("/**@param {(Object,string,null)} x\n" +
"@return {(Object,string)}*/function f(x) { return x; }");
}
public void testDefinePropertyOnNullableObject1() throws Exception {
testTypes("/** @type {Object} */ var n = {};\n" +
"/** @type {number} */ n.x = 1;\n" +
"/** @return {boolean} */function f() { return n.x; }",
"inconsistent return type\n" +
"found : number\n" +
"required: boolean");
}
public void testDefinePropertyOnNullableObject2() throws Exception {
testTypes("/** @constructor */ var T = function() {};\n" +
"/** @param {T} t\n@return {boolean} */function f(t) {\n" +
"t.x = 1; return t.x; }",
"inconsistent return type\n" +
"found : number\n" +
"required: boolean");
}
public void testUnknownConstructorInstanceType1() throws Exception {
testTypes("/** @return {Array} */ function g(f) { return new f(); }");
}
public void testUnknownConstructorInstanceType2() throws Exception {
testTypes("function g(f) { return /** @type Array */ new f(); }");
}
public void testUnknownConstructorInstanceType3() throws Exception {
testTypes("function g(f) { var x = new f(); x.a = 1; return x; }");
}
public void testUnknownPrototypeChain() throws Exception {
testTypes("/**\n" +
"* @param {Object} co\n" +
" * @return {Object}\n" +
" */\n" +
"function inst(co) {\n" +
" /** @constructor */\n" +
" var c = function() {};\n" +
" c.prototype = co.prototype;\n" +
" return new c;\n" +
"}");
}
public void testNamespacedConstructor() throws Exception {
Node root = parseAndTypeCheck(
"var goog = {};" +
"/** @constructor */ goog.MyClass = function() {};" +
"/** @return {!goog.MyClass} */ " +
"function foo() { return new goog.MyClass(); }");
JSType typeOfFoo = root.getLastChild().getJSType();
assert(typeOfFoo instanceof FunctionType);
JSType retType = ((FunctionType) typeOfFoo).getReturnType();
assert(retType instanceof ObjectType);
assertEquals("goog.MyClass", ((ObjectType) retType).getReferenceName());
}
public void testComplexNamespace() throws Exception {
String js =
"var goog = {};" +
"goog.foo = {};" +
"goog.foo.bar = 5;";
TypeCheckResult p = parseAndTypeCheckWithScope(js);
// goog type in the scope
JSType googScopeType = p.scope.getVar("goog").getType();
assertTrue(googScopeType instanceof ObjectType);
assertTrue("foo property not present on goog type",
((ObjectType) googScopeType).hasProperty("foo"));
assertFalse("bar property present on goog type",
((ObjectType) googScopeType).hasProperty("bar"));
// goog type on the VAR node
Node varNode = p.root.getFirstChild();
assertEquals(Token.VAR, varNode.getType());
JSType googNodeType = varNode.getFirstChild().getJSType();
assertTrue(googNodeType instanceof ObjectType);
// goog scope type and goog type on VAR node must be the same
assertTrue(googScopeType == googNodeType);
// goog type on the left of the GETPROP node (under fist ASSIGN)
Node getpropFoo1 = varNode.getNext().getFirstChild().getFirstChild();
assertEquals(Token.GETPROP, getpropFoo1.getType());
assertEquals("goog", getpropFoo1.getFirstChild().getString());
JSType googGetpropFoo1Type = getpropFoo1.getFirstChild().getJSType();
assertTrue(googGetpropFoo1Type instanceof ObjectType);
// still the same type as the one on the variable
assertTrue(googGetpropFoo1Type == googScopeType);
// the foo property should be defined on goog
JSType googFooType = ((ObjectType) googScopeType).getPropertyType("foo");
assertTrue(googFooType instanceof ObjectType);
// goog type on the left of the GETPROP lower level node
// (under second ASSIGN)
Node getpropFoo2 = varNode.getNext().getNext()
.getFirstChild().getFirstChild().getFirstChild();
assertEquals(Token.GETPROP, getpropFoo2.getType());
assertEquals("goog", getpropFoo2.getFirstChild().getString());
JSType googGetpropFoo2Type = getpropFoo2.getFirstChild().getJSType();
assertTrue(googGetpropFoo2Type instanceof ObjectType);
// still the same type as the one on the variable
assertTrue(googGetpropFoo2Type == googScopeType);
// goog.foo type on the left of the top level GETPROP node
// (under second ASSIGN)
JSType googFooGetprop2Type = getpropFoo2.getJSType();
assertTrue("goog.foo incorrectly annotated in goog.foo.bar selection",
googFooGetprop2Type instanceof ObjectType);
ObjectType googFooGetprop2ObjectType = (ObjectType) googFooGetprop2Type;
assertFalse("foo property present on goog.foo type",
googFooGetprop2ObjectType.hasProperty("foo"));
assertTrue("bar property not present on goog.foo type",
googFooGetprop2ObjectType.hasProperty("bar"));
assertEquals("bar property on goog.foo type incorrectly inferred",
NUMBER_TYPE, googFooGetprop2ObjectType.getPropertyType("bar"));
}
public void testAddingMethodsUsingPrototypeIdiomSimpleNamespace()
throws Exception {
Node js1Node = parseAndTypeCheck(
"/** @constructor */function A() {}" +
"A.prototype.m1 = 5");
ObjectType instanceType = getInstanceType(js1Node);
assertEquals(NATIVE_PROPERTIES_COUNT + 1,
instanceType.getPropertiesCount());
checkObjectType(instanceType, "m1", NUMBER_TYPE);
}
public void testAddingMethodsUsingPrototypeIdiomComplexNamespace1()
throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope(
"var goog = {};" +
"goog.A = /** @constructor */function() {};" +
"/** @type number */goog.A.prototype.m1 = 5");
testAddingMethodsUsingPrototypeIdiomComplexNamespace(p);
}
public void testAddingMethodsUsingPrototypeIdiomComplexNamespace2()
throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope(
"var goog = {};" +
"/** @constructor */goog.A = function() {};" +
"/** @type number */goog.A.prototype.m1 = 5");
testAddingMethodsUsingPrototypeIdiomComplexNamespace(p);
}
private void testAddingMethodsUsingPrototypeIdiomComplexNamespace(
TypeCheckResult p) {
ObjectType goog = (ObjectType) p.scope.getVar("goog").getType();
assertEquals(NATIVE_PROPERTIES_COUNT + 1, goog.getPropertiesCount());
JSType googA = goog.getPropertyType("A");
assertNotNull(googA);
assertTrue(googA instanceof FunctionType);
FunctionType googAFunction = (FunctionType) googA;
ObjectType classA = googAFunction.getInstanceType();
assertEquals(NATIVE_PROPERTIES_COUNT + 1, classA.getPropertiesCount());
checkObjectType(classA, "m1", NUMBER_TYPE);
}
public void testAddingMethodsPrototypeIdiomAndObjectLiteralSimpleNamespace()
throws Exception {
Node js1Node = parseAndTypeCheck(
"/** @constructor */function A() {}" +
"A.prototype = {m1: 5, m2: true}");
ObjectType instanceType = getInstanceType(js1Node);
assertEquals(NATIVE_PROPERTIES_COUNT + 2,
instanceType.getPropertiesCount());
checkObjectType(instanceType, "m1", NUMBER_TYPE);
checkObjectType(instanceType, "m2", BOOLEAN_TYPE);
}
public void testDontAddMethodsIfNoConstructor()
throws Exception {
Node js1Node = parseAndTypeCheck(
"function A() {}" +
"A.prototype = {m1: 5, m2: true}");
JSType functionAType = js1Node.getFirstChild().getJSType();
assertEquals("function (): undefined", functionAType.toString());
assertEquals(UNKNOWN_TYPE,
U2U_FUNCTION_TYPE.getPropertyType("m1"));
assertEquals(UNKNOWN_TYPE,
U2U_FUNCTION_TYPE.getPropertyType("m2"));
}
public void testFunctionAssignement() throws Exception {
testTypes("/**" +
"* @param {string} ph0" +
"* @param {string} ph1" +
"* @return {string}" +
"*/" +
"function MSG_CALENDAR_ACCESS_ERROR(ph0, ph1) {return ''}" +
"/** @type {Function} */" +
"var MSG_CALENDAR_ADD_ERROR = MSG_CALENDAR_ACCESS_ERROR;");
}
public void testAddMethodsPrototypeTwoWays() throws Exception {
Node js1Node = parseAndTypeCheck(
"/** @constructor */function A() {}" +
"A.prototype = {m1: 5, m2: true};" +
"A.prototype.m3 = 'third property!';");
ObjectType instanceType = getInstanceType(js1Node);
assertEquals("A", instanceType.toString());
assertEquals(NATIVE_PROPERTIES_COUNT + 3,
instanceType.getPropertiesCount());
checkObjectType(instanceType, "m1", NUMBER_TYPE);
checkObjectType(instanceType, "m2", BOOLEAN_TYPE);
checkObjectType(instanceType, "m3", STRING_TYPE);
}
public void testPrototypePropertyTypes() throws Exception {
Node js1Node = parseAndTypeCheck(
"/** @constructor */function A() {\n" +
" /** @type string */ this.m1;\n" +
" /** @type Object? */ this.m2 = {};\n" +
" /** @type boolean */ this.m3;\n" +
"}\n" +
"/** @type string */ A.prototype.m4;\n" +
"/** @type number */ A.prototype.m5 = 0;\n" +
"/** @type boolean */ A.prototype.m6;\n");
ObjectType instanceType = getInstanceType(js1Node);
assertEquals(NATIVE_PROPERTIES_COUNT + 6,
instanceType.getPropertiesCount());
checkObjectType(instanceType, "m1", STRING_TYPE);
checkObjectType(instanceType, "m2",
createUnionType(OBJECT_TYPE, NULL_TYPE));
checkObjectType(instanceType, "m3", BOOLEAN_TYPE);
checkObjectType(instanceType, "m4", STRING_TYPE);
checkObjectType(instanceType, "m5", NUMBER_TYPE);
checkObjectType(instanceType, "m6", BOOLEAN_TYPE);
}
public void testValueTypeBuiltInPrototypePropertyType() throws Exception {
Node node = parseAndTypeCheck("\"x\".charAt(0)");
assertEquals(STRING_TYPE, node.getFirstChild().getFirstChild().getJSType());
}
public void testDeclareBuiltInConstructor() throws Exception {
// Built-in prototype properties should be accessible
// even if the built-in constructor is declared.
Node node = parseAndTypeCheck(
"/** @constructor */ var String = function(opt_str) {};\n" +
"(new String(\"x\")).charAt(0)");
assertEquals(STRING_TYPE, node.getLastChild().getFirstChild().getJSType());
}
public void testExtendBuiltInType1() throws Exception {
String externs =
"/** @constructor */ var String = function(opt_str) {};\n" +
"/**\n" +
"* @param {number} start\n" +
"* @param {number} opt_length\n" +
"* @return {string}\n" +
"*/\n" +
"String.prototype.substr = function(start, opt_length) {};\n";
Node n1 = parseAndTypeCheck(externs + "(new String(\"x\")).substr(0,1);");
assertEquals(STRING_TYPE, n1.getLastChild().getFirstChild().getJSType());
}
public void testExtendBuiltInType2() throws Exception {
String externs =
"/** @constructor */ var String = function(opt_str) {};\n" +
"/**\n" +
"* @param {number} start\n" +
"* @param {number} opt_length\n" +
"* @return {string}\n" +
"*/\n" +
"String.prototype.substr = function(start, opt_length) {};\n";
Node n2 = parseAndTypeCheck(externs + "\"x\".substr(0,1);");
assertEquals(STRING_TYPE, n2.getLastChild().getFirstChild().getJSType());
}
public void testExtendFunction1() throws Exception {
Node n = parseAndTypeCheck("/**@return {number}*/Function.prototype.f = " +
"function() { return 1; };\n" +
"(new Function()).f();");
JSType type = n.getLastChild().getLastChild().getJSType();
assertEquals(NUMBER_TYPE, type);
}
public void testExtendFunction2() throws Exception {
Node n = parseAndTypeCheck("/**@return {number}*/Function.prototype.f = " +
"function() { return 1; };\n" +
"(function() {}).f();");
JSType type = n.getLastChild().getLastChild().getJSType();
assertEquals(NUMBER_TYPE, type);
}
public void testInheritanceCheck1() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"Sub.prototype.foo = function() {};");
}
public void testInheritanceCheck2() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override */Sub.prototype.foo = function() {};",
"property foo not defined on any superclass of Sub");
}
public void testInheritanceCheck3() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"Super.prototype.foo = function() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"Sub.prototype.foo = function() {};",
"property foo already defined on superclass Super; " +
"use @override to override it");
}
public void testInheritanceCheck4() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"Super.prototype.foo = function() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override */Sub.prototype.foo = function() {};");
}
public void testInheritanceCheck5() throws Exception {
testTypes(
"/** @constructor */function Root() {};" +
"Root.prototype.foo = function() {};" +
"/** @constructor\n @extends {Root} */function Super() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"Sub.prototype.foo = function() {};",
"property foo already defined on superclass Root; " +
"use @override to override it");
}
public void testInheritanceCheck6() throws Exception {
testTypes(
"/** @constructor */function Root() {};" +
"Root.prototype.foo = function() {};" +
"/** @constructor\n @extends {Root} */function Super() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override */Sub.prototype.foo = function() {};");
}
public void testInheritanceCheck7() throws Exception {
testTypes(
"var goog = {};" +
"/** @constructor */goog.Super = function() {};" +
"goog.Super.prototype.foo = 3;" +
"/** @constructor\n @extends {goog.Super} */goog.Sub = function() {};" +
"goog.Sub.prototype.foo = 5;",
"property foo already defined on superclass goog.Super; " +
"use @override to override it");
}
public void testInheritanceCheck8() throws Exception {
testTypes(
"var goog = {};" +
"/** @constructor */goog.Super = function() {};" +
"goog.Super.prototype.foo = 3;" +
"/** @constructor\n @extends {goog.Super} */goog.Sub = function() {};" +
"/** @override */goog.Sub.prototype.foo = 5;");
}
public void testInheritanceCheck9_1() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"Super.prototype.foo = function() { return 3; };" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override\n @return {number} */Sub.prototype.foo =\n" +
"function() { return 1; };");
}
public void testInheritanceCheck9_2() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"/** @return {number} */" +
"Super.prototype.foo = function() { return 1; };" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override */Sub.prototype.foo =\n" +
"function() {};");
}
public void testInheritanceCheck9_3() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"/** @return {number} */" +
"Super.prototype.foo = function() { return 1; };" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override\n @return {string} */Sub.prototype.foo =\n" +
"function() { return \"some string\" };",
"mismatch of the foo property type and the type of the property it " +
"overrides from superclass Super\n" +
"original: function (this:Super): number\n" +
"override: function (this:Sub): string");
}
public void testInheritanceCheck10_1() throws Exception {
testTypes(
"/** @constructor */function Root() {};" +
"Root.prototype.foo = function() { return 3; };" +
"/** @constructor\n @extends {Root} */function Super() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override\n @return {number} */Sub.prototype.foo =\n" +
"function() { return 1; };");
}
public void testInheritanceCheck10_2() throws Exception {
testTypes(
"/** @constructor */function Root() {};" +
"/** @return {number} */" +
"Root.prototype.foo = function() { return 1; };" +
"/** @constructor\n @extends {Root} */function Super() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override */Sub.prototype.foo =\n" +
"function() {};");
}
public void testInheritanceCheck10_3() throws Exception {
testTypes(
"/** @constructor */function Root() {};" +
"/** @return {number} */" +
"Root.prototype.foo = function() { return 1; };" +
"/** @constructor\n @extends {Root} */function Super() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override\n @return {string} */Sub.prototype.foo =\n" +
"function() { return \"some string\" };",
"mismatch of the foo property type and the type of the property it " +
"overrides from superclass Root\n" +
"original: function (this:Root): number\n" +
"override: function (this:Sub): string");
}
public void testInterfaceInheritanceCheck11() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"/** @param {number} bar */Super.prototype.foo = function(bar) {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override\n @param {string} bar */Sub.prototype.foo =\n" +
"function(bar) {};",
"mismatch of the foo property type and the type of the property it " +
"overrides from superclass Super\n" +
"original: function (this:Super, number): undefined\n" +
"override: function (this:Sub, string): undefined");
}
public void testInheritanceCheck12() throws Exception {
testTypes(
"var goog = {};" +
"/** @constructor */goog.Super = function() {};" +
"goog.Super.prototype.foo = 3;" +
"/** @constructor\n @extends {goog.Super} */goog.Sub = function() {};" +
"/** @override */goog.Sub.prototype.foo = \"some string\";",
"mismatch of the foo property type and the type of the property it " +
"overrides from superclass goog.Super\n" +
"original: number\n" +
"override: string");
}
public void testInheritanceCheck13() throws Exception {
testTypes(
"var goog = {};\n" +
"/** @constructor\n @extends {goog.Missing} */function Sub() {};" +
"/** @override */Sub.prototype.foo = function() {};",
"Bad type annotation. Unknown type goog.Missing");
}
public void testInheritanceCheck14() throws Exception {
testClosureTypes(
"var goog = {};\n" +
"/** @constructor\n @extends {goog.Missing} */\n" +
"goog.Super = function() {};\n" +
"/** @constructor\n @extends {goog.Super} */function Sub() {};" +
"/** @override */Sub.prototype.foo = function() {};",
"Bad type annotation. Unknown type goog.Missing");
}
public void testInheritanceCheck15() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"/** @param {number} bar */Super.prototype.foo;" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override\n @param {number} bar */Sub.prototype.foo =\n" +
"function(bar) {};");
}
public void testInheritanceCheck16() throws Exception {
testTypes(
"var goog = {};" +
"/** @constructor */goog.Super = function() {};" +
"/** @type {number} */ goog.Super.prototype.foo = 3;" +
"/** @constructor\n @extends {goog.Super} */goog.Sub = function() {};" +
"/** @type {number} */ goog.Sub.prototype.foo = 5;",
"property foo already defined on superclass goog.Super; " +
"use @override to override it");
}
public void testInterfacePropertyOverride1() throws Exception {
testTypes(
"/** @interface */function Super() {};" +
"/** @desc description */Super.prototype.foo = function() {};" +
"/** @interface\n @extends {Super} */function Sub() {};" +
"/** @desc description */Sub.prototype.foo = function() {};");
}
public void testInterfacePropertyOverride2() throws Exception {
testTypes(
"/** @interface */function Root() {};" +
"/** @desc description */Root.prototype.foo = function() {};" +
"/** @interface\n @extends {Root} */function Super() {};" +
"/** @interface\n @extends {Super} */function Sub() {};" +
"/** @desc description */Sub.prototype.foo = function() {};");
}
public void testInterfaceInheritanceCheck1() throws Exception {
testTypes(
"/** @interface */function Super() {};" +
"/** @desc description */Super.prototype.foo = function() {};" +
"/** @constructor\n @implements {Super} */function Sub() {};" +
"Sub.prototype.foo = function() {};",
"property foo already defined on interface Super; use @override to " +
"override it");
}
public void testInterfaceInheritanceCheck2() throws Exception {
testTypes(
"/** @interface */function Super() {};" +
"/** @desc description */Super.prototype.foo = function() {};" +
"/** @constructor\n @implements {Super} */function Sub() {};" +
"/** @override */Sub.prototype.foo = function() {};");
}
public void testInterfaceInheritanceCheck3() throws Exception {
testTypes(
"/** @interface */function Root() {};" +
"/** @return {number} */Root.prototype.foo = function() {};" +
"/** @interface\n @extends {Root} */function Super() {};" +
"/** @constructor\n @implements {Super} */function Sub() {};" +
"/** @return {number} */Sub.prototype.foo = function() { return 1;};",
"property foo already defined on interface Root; use @override to " +
"override it");
}
public void testInterfaceInheritanceCheck4() throws Exception {
testTypes(
"/** @interface */function Root() {};" +
"/** @return {number} */Root.prototype.foo = function() {};" +
"/** @interface\n @extends {Root} */function Super() {};" +
"/** @constructor\n @implements {Super} */function Sub() {};" +
"/** @override\n * @return {number} */Sub.prototype.foo =\n" +
"function() { return 1;};");
}
public void testInterfaceInheritanceCheck5() throws Exception {
testTypes(
"/** @interface */function Super() {};" +
"/** @return {string} */Super.prototype.foo = function() {};" +
"/** @constructor\n @implements {Super} */function Sub() {};" +
"/** @override\n @return {number} */Sub.prototype.foo =\n" +
"function() { return 1; };",
"mismatch of the foo property type and the type of the property it " +
"overrides from interface Super\n" +
"original: function (this:Super): string\n" +
"override: function (this:Sub): number");
}
public void testInterfaceInheritanceCheck6() throws Exception {
testTypes(
"/** @interface */function Root() {};" +
"/** @return {string} */Root.prototype.foo = function() {};" +
"/** @interface\n @extends {Root} */function Super() {};" +
"/** @constructor\n @implements {Super} */function Sub() {};" +
"/** @override\n @return {number} */Sub.prototype.foo =\n" +
"function() { return 1; };",
"mismatch of the foo property type and the type of the property it " +
"overrides from interface Root\n" +
"original: function (this:Root): string\n" +
"override: function (this:Sub): number");
}
public void testInterfaceInheritanceCheck7() throws Exception {
testTypes(
"/** @interface */function Super() {};" +
"/** @param {number} bar */Super.prototype.foo = function(bar) {};" +
"/** @constructor\n @implements {Super} */function Sub() {};" +
"/** @override\n @param {string} bar */Sub.prototype.foo =\n" +
"function(bar) {};",
"mismatch of the foo property type and the type of the property it " +
"overrides from interface Super\n" +
"original: function (this:Super, number): undefined\n" +
"override: function (this:Sub, string): undefined");
}
public void testInterfaceInheritanceCheck8() throws Exception {
testTypes(
"/** @constructor\n @implements {Super} */function Sub() {};" +
"/** @override */Sub.prototype.foo = function() {};",
new String[] {
"Bad type annotation. Unknown type Super",
"property foo not defined on any superclass of Sub"
});
}
public void testInterfaceInheritanceCheck9() throws Exception {
testTypes(
"/** @interface */ function I() {}" +
"/** @return {number} */ I.prototype.bar = function() {};" +
"/** @constructor */ function F() {}" +
"/** @return {number} */ F.prototype.bar = function() {return 3; };" +
"/** @return {number} */ F.prototype.foo = function() {return 3; };" +
"/** @constructor \n * @extends {F} \n * @implements {I} */ " +
"function G() {}" +
"/** @return {string} */ function f() { return new G().bar(); }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testInterfaceInheritanceCheck10() throws Exception {
testTypes(
"/** @interface */ function I() {}" +
"/** @return {number} */ I.prototype.bar = function() {};" +
"/** @constructor */ function F() {}" +
"/** @return {number} */ F.prototype.foo = function() {return 3; };" +
"/** @constructor \n * @extends {F} \n * @implements {I} */ " +
"function G() {}" +
"/** @return {number} \n * @override */ " +
"G.prototype.bar = G.prototype.foo;" +
"/** @return {string} */ function f() { return new G().bar(); }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testInterfaceInheritanceCheck12() throws Exception {
testTypes(
"/** @interface */ function I() {};\n" +
"/** @type {string} */ I.prototype.foobar;\n" +
"/** \n * @constructor \n * @implements {I} */\n" +
"function C() {\n" +
"/** \n * @type {number} */ this.foobar = 2;};\n" +
"/** @type {I} */ \n var test = new C(); alert(test.foobar);",
"mismatch of the foobar property type and the type of the property" +
" it overrides from interface I\n" +
"original: string\n" +
"override: number");
}
public void testInterfaceInheritanceCheck13() throws Exception {
testTypes(
"function abstractMethod() {};\n" +
"/** @interface */var base = function() {};\n" +
"/** @extends {base} \n @interface */ var Int = function() {}\n" +
"/** @type {{bar : !Function}} */ var x; \n" +
"/** @type {!Function} */ base.prototype.bar = abstractMethod; \n" +
"/** @type {Int} */ foo;\n" +
"foo.bar();");
}
public void testInterfacePropertyNotImplemented() throws Exception {
testTypes(
"/** @interface */function Int() {};" +
"/** @desc description */Int.prototype.foo = function() {};" +
"/** @constructor\n @implements {Int} */function Foo() {};",
"property foo on interface Int is not implemented by type Foo");
}
public void testInterfacePropertyNotImplemented2() throws Exception {
testTypes(
"/** @interface */function Int() {};" +
"/** @desc description */Int.prototype.foo = function() {};" +
"/** @interface \n @extends {Int} */function Int2() {};" +
"/** @constructor\n @implements {Int2} */function Foo() {};",
"property foo on interface Int is not implemented by type Foo");
}
public void testStubConstructorImplementingInterface() throws Exception {
// This does not throw a warning for unimplemented property because Foo is
// just a stub.
testTypes(
// externs
"/** @interface */ function Int() {}\n" +
"/** @desc description */Int.prototype.foo = function() {};" +
"/** @constructor \n @implements {Int} */ var Foo;\n",
"", null, false);
}
public void testObjectLiteral() throws Exception {
Node n = parseAndTypeCheck("var a = {m1: 7, m2: 'hello'}");
Node nameNode = n.getFirstChild().getFirstChild();
Node objectNode = nameNode.getFirstChild();
// node extraction
assertEquals(Token.NAME, nameNode.getType());
assertEquals(Token.OBJECTLIT, objectNode.getType());
// value's type
ObjectType objectType =
(ObjectType) objectNode.getJSType();
assertEquals(NUMBER_TYPE, objectType.getPropertyType("m1"));
assertEquals(STRING_TYPE, objectType.getPropertyType("m2"));
// variable's type
assertEquals(objectType, nameNode.getJSType());
}
public void testObjectLiteralDeclaration1() throws Exception {
testTypes(
"var x = {" +
"/** @type {boolean} */ abc: true," +
"/** @type {number} */ 'def': 0," +
"/** @type {string} */ 3: 'fgh'" +
"};");
}
public void testObjectLiteralDeclaration2() throws Exception {
testTypes(
"var x = {" +
" /** @type {boolean} */ abc: true" +
"};" +
"x.abc = 0;",
"assignment to property abc of x\n" +
"found : number\n" +
"required: boolean");
}
public void testObjectLiteralDeclaration3() throws Exception {
testTypes(
"/** @param {{foo: !Function}} x */ function f(x) {}" +
"f({foo: function() {}});");
}
public void testObjectLiteralDeclaration4() throws Exception {
testClosureTypes(
"var x = {" +
" /** @param {boolean} x */ abc: function(x) {}" +
"};" +
"/**\n" +
" * @param {string} x\n" +
" * @suppress {duplicate}\n" +
" */ x.abc = function(x) {};",
"assignment to property abc of x\n" +
"found : function (string): undefined\n" +
"required: function (boolean): undefined");
// TODO(user): suppress {duplicate} currently also silence the
// redefining type error in the TypeValidator. May be it needs
// a new suppress name instead?
}
public void testObjectLiteralDeclaration5() throws Exception {
testTypes(
"var x = {" +
" /** @param {boolean} x */ abc: function(x) {}" +
"};" +
"/**\n" +
" * @param {boolean} x\n" +
" * @suppress {duplicate}\n" +
" */ x.abc = function(x) {};");
}
public void testObjectLiteralDeclaration6() throws Exception {
testTypes(
"var x = {};" +
"/**\n" +
" * @param {boolean} x\n" +
" * @suppress {duplicate}\n" +
" */ x.abc = function(x) {};" +
"x = {" +
" /**\n" +
" * @param {boolean} x\n" +
" * @suppress {duplicate}\n" +
" */" +
" abc: function(x) {}" +
"};");
}
public void testObjectLiteralDeclaration7() throws Exception {
testTypes(
"var x = {};" +
"/**\n" +
" * @type {function(boolean): undefined}\n" +
" */ x.abc = function(x) {};" +
"x = {" +
" /**\n" +
" * @param {boolean} x\n" +
" * @suppress {duplicate}\n" +
" */" +
" abc: function(x) {}" +
"};");
}
public void testCallDateConstructorAsFunction() throws Exception {
// ECMA-262 15.9.2: When Date is called as a function rather than as a
// constructor, it returns a string.
Node n = parseAndTypeCheck("Date()");
assertEquals(STRING_TYPE, n.getFirstChild().getFirstChild().getJSType());
}
// According to ECMA-262, Error & Array function calls are equivalent to
// constructor calls.
public void testCallErrorConstructorAsFunction() throws Exception {
Node n = parseAndTypeCheck("Error('x')");
assertEquals(ERROR_TYPE,
n.getFirstChild().getFirstChild().getJSType());
}
public void testCallArrayConstructorAsFunction() throws Exception {
Node n = parseAndTypeCheck("Array()");
assertEquals(ARRAY_TYPE,
n.getFirstChild().getFirstChild().getJSType());
}
public void testPropertyTypeOfUnionType() throws Exception {
testTypes("var a = {};" +
"/** @constructor */ a.N = function() {};\n" +
"a.N.prototype.p = 1;\n" +
"/** @constructor */ a.S = function() {};\n" +
"a.S.prototype.p = 'a';\n" +
"/** @param {!a.N|!a.S} x\n@return {string} */\n" +
"var f = function(x) { return x.p; };",
"inconsistent return type\n" +
"found : (number|string)\n" +
"required: string");
}
// TODO(user): We should flag these as invalid. This will probably happen
// when we make sure the interface is never referenced outside of its
// definition. We might want more specific and helpful error messages.
//public void testWarningOnInterfacePrototype() throws Exception {
// testTypes("/** @interface */ u.T = function() {};\n" +
// "/** @return {number} */ u.T.prototype = function() { };",
// "e of its definition");
//}
//
//public void testBadPropertyOnInterface1() throws Exception {
// testTypes("/** @interface */ u.T = function() {};\n" +
// "/** @return {number} */ u.T.f = function() { return 1;};",
// "cannot reference an interface ouside of its definition");
//}
//
//public void testBadPropertyOnInterface2() throws Exception {
// testTypes("/** @interface */ function T() {};\n" +
// "/** @return {number} */ T.f = function() { return 1;};",
// "cannot reference an interface ouside of its definition");
//}
//
//public void testBadPropertyOnInterface3() throws Exception {
// testTypes("/** @interface */ u.T = function() {}; u.T.x",
// "cannot reference an interface ouside of its definition");
//}
//
//public void testBadPropertyOnInterface4() throws Exception {
// testTypes("/** @interface */ function T() {}; T.x;",
// "cannot reference an interface ouside of its definition");
//}
public void testAnnotatedPropertyOnInterface1() throws Exception {
// For interfaces we must allow function definitions that don't have a
// return statement, even though they declare a returned type.
testTypes("/** @interface */ u.T = function() {};\n" +
"/** @return {number} */ u.T.prototype.f = function() {};");
}
public void testAnnotatedPropertyOnInterface2() throws Exception {
testTypes("/** @interface */ u.T = function() {};\n" +
"/** @return {number} */ u.T.prototype.f = function() { };");
}
public void testAnnotatedPropertyOnInterface3() throws Exception {
testTypes("/** @interface */ function T() {};\n" +
"/** @return {number} */ T.prototype.f = function() { };");
}
public void testAnnotatedPropertyOnInterface4() throws Exception {
testTypes(
CLOSURE_DEFS +
"/** @interface */ function T() {};\n" +
"/** @return {number} */ T.prototype.f = goog.abstractMethod;");
}
// TODO(user): If we want to support this syntax we have to warn about
// missing annotations.
//public void testWarnUnannotatedPropertyOnInterface1() throws Exception {
// testTypes("/** @interface */ u.T = function () {}; u.T.prototype.x;",
// "interface property x is not annotated");
//}
//
//public void testWarnUnannotatedPropertyOnInterface2() throws Exception {
// testTypes("/** @interface */ function T() {}; T.prototype.x;",
// "interface property x is not annotated");
//}
public void testWarnUnannotatedPropertyOnInterface5() throws Exception {
testTypes("/** @interface */ u.T = function () {};\n" +
"/** @desc x does something */u.T.prototype.x = function() {};");
}
public void testWarnUnannotatedPropertyOnInterface6() throws Exception {
testTypes("/** @interface */ function T() {};\n" +
"/** @desc x does something */T.prototype.x = function() {};");
}
// TODO(user): If we want to support this syntax we have to warn about
// the invalid type of the interface member.
//public void testWarnDataPropertyOnInterface1() throws Exception {
// testTypes("/** @interface */ u.T = function () {};\n" +
// "/** @type {number} */u.T.prototype.x;",
// "interface members can only be plain functions");
//}
public void testDataPropertyOnInterface1() throws Exception {
testTypes("/** @interface */ function T() {};\n" +
"/** @type {number} */T.prototype.x;");
}
public void testDataPropertyOnInterface2() throws Exception {
reportMissingOverrides = CheckLevel.OFF;
testTypes("/** @interface */ function T() {};\n" +
"/** @type {number} */T.prototype.x;\n" +
"/** @constructor \n" +
" * @implements {T} \n" +
" */\n" +
"function C() {}\n" +
"C.prototype.x = 'foo';",
"mismatch of the x property type and the type of the property it " +
"overrides from interface T\n" +
"original: number\n" +
"override: string");
}
public void testDataPropertyOnInterface3() throws Exception {
testTypes("/** @interface */ function T() {};\n" +
"/** @type {number} */T.prototype.x;\n" +
"/** @constructor \n" +
" * @implements {T} \n" +
" */\n" +
"function C() {}\n" +
"/** @override */\n" +
"C.prototype.x = 'foo';",
"mismatch of the x property type and the type of the property it " +
"overrides from interface T\n" +
"original: number\n" +
"override: string");
}
public void testDataPropertyOnInterface4() throws Exception {
testTypes("/** @interface */ function T() {};\n" +
"/** @type {number} */T.prototype.x;\n" +
"/** @constructor \n" +
" * @implements {T} \n" +
" */\n" +
"function C() { /** @type {string} */ \n this.x = 'foo'; }\n",
"mismatch of the x property type and the type of the property it " +
"overrides from interface T\n" +
"original: number\n" +
"override: string");
}
public void testWarnDataPropertyOnInterface3() throws Exception {
testTypes("/** @interface */ u.T = function () {};\n" +
"/** @type {number} */u.T.prototype.x = 1;",
"interface members can only be empty property declarations, "
+ "empty functions, or goog.abstractMethod");
}
public void testWarnDataPropertyOnInterface4() throws Exception {
testTypes("/** @interface */ function T() {};\n" +
"/** @type {number} */T.prototype.x = 1;",
"interface members can only be empty property declarations, "
+ "empty functions, or goog.abstractMethod");
}
// TODO(user): If we want to support this syntax we should warn about the
// mismatching types in the two tests below.
//public void testErrorMismatchingPropertyOnInterface1() throws Exception {
// testTypes("/** @interface */ u.T = function () {};\n" +
// "/** @param {Number} foo */u.T.prototype.x =\n" +
// "/** @param {String} foo */function(foo) {};",
// "found : \n" +
// "required: ");
//}
//
//public void testErrorMismatchingPropertyOnInterface2() throws Exception {
// testTypes("/** @interface */ function T() {};\n" +
// "/** @return {number} */T.prototype.x =\n" +
// "/** @return {string} */function() {};",
// "found : \n" +
// "required: ");
//}
// TODO(user): We should warn about this (bar is missing an annotation). We
// probably don't want to warn about all missing parameter annotations, but
// we should be as strict as possible regarding interfaces.
//public void testErrorMismatchingPropertyOnInterface3() throws Exception {
// testTypes("/** @interface */ u.T = function () {};\n" +
// "/** @param {Number} foo */u.T.prototype.x =\n" +
// "function(foo, bar) {};",
// "found : \n" +
// "required: ");
//}
public void testErrorMismatchingPropertyOnInterface4() throws Exception {
testTypes("/** @interface */ u.T = function () {};\n" +
"/** @param {Number} foo */u.T.prototype.x =\n" +
"function() {};",
"parameter foo does not appear in u.T.prototype.x's parameter list");
}
public void testErrorMismatchingPropertyOnInterface5() throws Exception {
testTypes("/** @interface */ function T() {};\n" +
"/** @type {number} */T.prototype.x = function() { };",
"assignment to property x of T.prototype\n" +
"found : function (): undefined\n" +
"required: number");
}
public void testErrorMismatchingPropertyOnInterface6() throws Exception {
testClosureTypesMultipleWarnings(
"/** @interface */ function T() {};\n" +
"/** @return {number} */T.prototype.x = 1",
Lists.newArrayList(
"assignment to property x of T.prototype\n" +
"found : number\n" +
"required: function (this:T): number",
"interface members can only be empty property declarations, " +
"empty functions, or goog.abstractMethod"));
}
public void testInterfaceNonEmptyFunction() throws Exception {
testTypes("/** @interface */ function T() {};\n" +
"T.prototype.x = function() { return 'foo'; }",
"interface member functions must have an empty body"
);
}
public void testDoubleNestedInterface() throws Exception {
testTypes("/** @interface */ var I1 = function() {};\n" +
"/** @interface */ I1.I2 = function() {};\n" +
"/** @interface */ I1.I2.I3 = function() {};\n");
}
public void testStaticDataPropertyOnNestedInterface() throws Exception {
testTypes("/** @interface */ var I1 = function() {};\n" +
"/** @interface */ I1.I2 = function() {};\n" +
"/** @type {number} */ I1.I2.x = 1;\n");
}
public void testInterfaceInstantiation() throws Exception {
testTypes("/** @interface */var f = function(){}; new f",
"cannot instantiate non-constructor");
}
public void testPrototypeLoop() throws Exception {
testClosureTypesMultipleWarnings(
suppressMissingProperty("foo") +
"/** @constructor \n * @extends {T} */var T = function() {};" +
"alert((new T).foo);",
Lists.newArrayList(
"Parse error. Cycle detected in inheritance chain of type T",
"Could not resolve type in @extends tag of T"));
}
public void testDirectPrototypeAssign() throws Exception {
// For now, we just ignore @type annotations on the prototype.
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @constructor */ function Bar() {}" +
"/** @type {Array} */ Bar.prototype = new Foo()");
}
// In all testResolutionViaRegistry* tests, since u is unknown, u.T can only
// be resolved via the registry and not via properties.
public void testResolutionViaRegistry1() throws Exception {
testTypes("/** @constructor */ u.T = function() {};\n" +
"/** @type {(number|string)} */ u.T.prototype.a;\n" +
"/**\n" +
"* @param {u.T} t\n" +
"* @return {string}\n" +
"*/\n" +
"var f = function(t) { return t.a; };",
"inconsistent return type\n" +
"found : (number|string)\n" +
"required: string");
}
public void testResolutionViaRegistry2() throws Exception {
testTypes(
"/** @constructor */ u.T = function() {" +
" this.a = 0; };\n" +
"/**\n" +
"* @param {u.T} t\n" +
"* @return {string}\n" +
"*/\n" +
"var f = function(t) { return t.a; };",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testResolutionViaRegistry3() throws Exception {
testTypes("/** @constructor */ u.T = function() {};\n" +
"/** @type {(number|string)} */ u.T.prototype.a = 0;\n" +
"/**\n" +
"* @param {u.T} t\n" +
"* @return {string}\n" +
"*/\n" +
"var f = function(t) { return t.a; };",
"inconsistent return type\n" +
"found : (number|string)\n" +
"required: string");
}
public void testResolutionViaRegistry4() throws Exception {
testTypes("/** @constructor */ u.A = function() {};\n" +
"/**\n* @constructor\n* @extends {u.A}\n*/\nu.A.A = function() {}\n;" +
"/**\n* @constructor\n* @extends {u.A}\n*/\nu.A.B = function() {};\n" +
"var ab = new u.A.B();\n" +
"/** @type {!u.A} */ var a = ab;\n" +
"/** @type {!u.A.A} */ var aa = ab;\n",
"initializing variable\n" +
"found : u.A.B\n" +
"required: u.A.A");
}
public void testResolutionViaRegistry5() throws Exception {
Node n = parseAndTypeCheck("/** @constructor */ u.T = function() {}; u.T");
JSType type = n.getLastChild().getLastChild().getJSType();
assertFalse(type.isUnknownType());
assertTrue(type instanceof FunctionType);
assertEquals("u.T",
((FunctionType) type).getInstanceType().getReferenceName());
}
public void testGatherProperyWithoutAnnotation1() throws Exception {
Node n = parseAndTypeCheck("/** @constructor */ var T = function() {};" +
"/** @type {!T} */var t; t.x; t;");
JSType type = n.getLastChild().getLastChild().getJSType();
assertFalse(type.isUnknownType());
assertTrue(type instanceof ObjectType);
ObjectType objectType = (ObjectType) type;
assertFalse(objectType.hasProperty("x"));
assertEquals(
Lists.newArrayList(objectType),
registry.getTypesWithProperty("x"));
}
public void testGatherProperyWithoutAnnotation2() throws Exception {
TypeCheckResult ns =
parseAndTypeCheckWithScope("/** @type {!Object} */var t; t.x; t;");
Node n = ns.root;
Scope s = ns.scope;
JSType type = n.getLastChild().getLastChild().getJSType();
assertFalse(type.isUnknownType());
assertEquals(type, OBJECT_TYPE);
assertTrue(type instanceof ObjectType);
ObjectType objectType = (ObjectType) type;
assertFalse(objectType.hasProperty("x"));
assertEquals(
Lists.newArrayList(OBJECT_TYPE),
registry.getTypesWithProperty("x"));
}
public void testFunctionMasksVariableBug() throws Exception {
testTypes("var x = 4; var f = function x(b) { return b ? 1 : x(true); };",
"function x masks variable (IE bug)");
}
public void testDfa1() throws Exception {
testTypes("var x = null;\n x = 1;\n /** @type number */ var y = x;");
}
public void testDfa2() throws Exception {
testTypes("function u() {}\n" +
"/** @return {number} */ function f() {\nvar x = 'todo';\n" +
"if (u()) { x = 1; } else { x = 2; } return x;\n}");
}
public void testDfa3() throws Exception {
testTypes("function u() {}\n" +
"/** @return {number} */ function f() {\n" +
"/** @type {number|string} */ var x = 'todo';\n" +
"if (u()) { x = 1; } else { x = 2; } return x;\n}");
}
public void testDfa4() throws Exception {
testTypes("/** @param {Date?} d */ function f(d) {\n" +
"if (!d) { return; }\n" +
"/** @type {!Date} */ var e = d;\n}");
}
public void testDfa5() throws Exception {
testTypes("/** @return {string?} */ function u() {return 'a';}\n" +
"/** @param {string?} x\n@return {string} */ function f(x) {\n" +
"while (!x) { x = u(); }\nreturn x;\n}");
}
public void testDfa6() throws Exception {
testTypes("/** @return {Object?} */ function u() {return {};}\n" +
"/** @param {Object?} x */ function f(x) {\n" +
"while (x) { x = u(); if (!x) { x = u(); } }\n}");
}
public void testDfa7() throws Exception {
testTypes("/** @constructor */ var T = function() {};\n" +
"/** @type {Date?} */ T.prototype.x = null;\n" +
"/** @param {!T} t */ function f(t) {\n" +
"if (!t.x) { return; }\n" +
"/** @type {!Date} */ var e = t.x;\n}");
}
public void testDfa8() throws Exception {
testTypes("/** @constructor */ var T = function() {};\n" +
"/** @type {number|string} */ T.prototype.x = '';\n" +
"function u() {}\n" +
"/** @param {!T} t\n@return {number} */ function f(t) {\n" +
"if (u()) { t.x = 1; } else { t.x = 2; } return t.x;\n}");
}
public void testDfa9() throws Exception {
testTypes("function f() {\n/** @type {string?} */var x;\nx = null;\n" +
"if (x == null) { return 0; } else { return 1; } }",
"condition always evaluates to true\n" +
"left : null\n" +
"right: null");
}
public void testDfa10() throws Exception {
testTypes("/** @param {null} x */ function g(x) {}" +
"/** @param {string?} x */function f(x) {\n" +
"if (!x) { x = ''; }\n" +
"if (g(x)) { return 0; } else { return 1; } }",
"actual parameter 1 of g does not match formal parameter\n" +
"found : string\n" +
"required: null");
}
public void testDfa11() throws Exception {
testTypes("/** @param {string} opt_x\n@return {string} */\n" +
"function f(opt_x) { if (!opt_x) { " +
"throw new Error('x cannot be empty'); } return opt_x; }");
}
public void testDfa12() throws Exception {
testTypes("/** @param {string} x \n * @constructor \n */" +
"var Bar = function(x) {};" +
"/** @param {string} x */ function g(x) { return true; }" +
"/** @param {string|number} opt_x */ " +
"function f(opt_x) { " +
" if (opt_x) { new Bar(g(opt_x) && 'x'); }" +
"}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : (number|string)\n" +
"required: string");
}
public void testDfa13() throws Exception {
testTypes(
"/**\n" +
" * @param {string} x \n" +
" * @param {number} y \n" +
" * @param {number} z \n" +
" */" +
"function g(x, y, z) {}" +
"function f() { " +
" var x = 'a'; g(x, x = 3, x);" +
"}");
}
public void testTypeInferenceWithCast1() throws Exception {
testTypes(
"/**@return {(number,null,undefined)}*/function u(x) {return null;}" +
"/**@param {number?} x\n@return {number?}*/function f(x) {return x;}" +
"/**@return {number?}*/function g(x) {" +
"var y = /**@type {number?}*/(u(x)); return f(y);}");
}
public void testTypeInferenceWithCast2() throws Exception {
testTypes(
"/**@return {(number,null,undefined)}*/function u(x) {return null;}" +
"/**@param {number?} x\n@return {number?}*/function f(x) {return x;}" +
"/**@return {number?}*/function g(x) {" +
"var y; y = /**@type {number?}*/(u(x)); return f(y);}");
}
public void testTypeInferenceWithCast3() throws Exception {
testTypes(
"/**@return {(number,null,undefined)}*/function u(x) {return 1;}" +
"/**@return {number}*/function g(x) {" +
"return /**@type {number}*/(u(x));}");
}
public void testTypeInferenceWithCast4() throws Exception {
testTypes(
"/**@return {(number,null,undefined)}*/function u(x) {return 1;}" +
"/**@return {number}*/function g(x) {" +
"return /**@type {number}*/(u(x)) && 1;}");
}
public void testTypeInferenceWithCast5() throws Exception {
testTypes(
"/** @param {number} x */ function foo(x) {}" +
"/** @param {{length:*}} y */ function bar(y) {" +
" /** @type {string} */ y.length;" +
" foo(y.length);" +
"}",
"actual parameter 1 of foo does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testTypeInferenceWithClosure1() throws Exception {
testTypes(
"/** @return {boolean} */" +
"function f() {" +
" /** @type {?string} */ var x = null;" +
" function g() { x = 'y'; } g(); " +
" return x == null;" +
"}");
}
public void testTypeInferenceWithClosure2() throws Exception {
testTypes(
"/** @return {boolean} */" +
"function f() {" +
" /** @type {?string} */ var x = null;" +
" function g() { x = 'y'; } g(); " +
" return x === 3;" +
"}",
"condition always evaluates to the same value\n" +
"left : (null|string)\n" +
"right: number");
}
public void testTypeInferenceWithNoEntry1() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"/** @constructor */ function Foo() {}" +
"Foo.prototype.init = function() {" +
" /** @type {?{baz: number}} */ this.bar = {baz: 3};" +
"};" +
"/**\n" +
" * @extends {Foo}\n" +
" * @constructor\n" +
" */" +
"function SubFoo() {}" +
"/** Method */" +
"SubFoo.prototype.method = function() {" +
" for (var i = 0; i < 10; i++) {" +
" f(this.bar);" +
" f(this.bar.baz);" +
" }" +
"};",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (null|{baz: number})\n" +
"required: number");
}
public void testTypeInferenceWithNoEntry2() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @param {number} x */ function f(x) {}" +
"/** @param {!Object} x */ function g(x) {}" +
"/** @constructor */ function Foo() {}" +
"Foo.prototype.init = function() {" +
" /** @type {?{baz: number}} */ this.bar = {baz: 3};" +
"};" +
"/**\n" +
" * @extends {Foo}\n" +
" * @constructor\n" +
" */" +
"function SubFoo() {}" +
"/** Method */" +
"SubFoo.prototype.method = function() {" +
" for (var i = 0; i < 10; i++) {" +
" f(this.bar);" +
" goog.asserts.assert(this.bar);" +
" g(this.bar);" +
" }" +
"};",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (null|{baz: number})\n" +
"required: number");
}
public void testForwardPropertyReference() throws Exception {
testTypes("/** @constructor */ var Foo = function() { this.init(); };" +
"/** @return {string} */" +
"Foo.prototype.getString = function() {" +
" return this.number_;" +
"};" +
"Foo.prototype.init = function() {" +
" /** @type {number} */" +
" this.number_ = 3;" +
"};",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testNoForwardTypeDeclaration() throws Exception {
testTypes(
"/** @param {MyType} x */ function f(x) {}",
"Bad type annotation. Unknown type MyType");
}
public void testNoForwardTypeDeclarationAndNoBraces() throws Exception {
testTypes("/** @return The result. */ function f() {}");
}
public void testForwardTypeDeclaration1() throws Exception {
testClosureTypes(
// malformed addDependency calls shouldn't cause a crash
"goog.addDependency();" +
"goog.addDependency('y', [goog]);" +
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/** @param {MyType} x \n * @return {number} */" +
"function f(x) { return 3; }", null);
}
public void testForwardTypeDeclaration2() throws Exception {
String f = "goog.addDependency('zzz.js', ['MyType'], []);" +
"/** @param {MyType} x */ function f(x) { }";
testClosureTypes(f, null);
testClosureTypes(f + "f(3);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: (MyType|null)");
}
public void testForwardTypeDeclaration3() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/** @param {MyType} x */ function f(x) { return x; }" +
"/** @constructor */ var MyType = function() {};" +
"f(3);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: (MyType|null)");
}
public void testForwardTypeDeclaration4() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/** @param {MyType} x */ function f(x) { return x; }" +
"/** @constructor */ var MyType = function() {};" +
"f(new MyType());",
null);
}
public void testForwardTypeDeclaration5() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/**\n" +
" * @constructor\n" +
" * @extends {MyType}\n" +
" */ var YourType = function() {};" +
"/** @override */ YourType.prototype.method = function() {};",
"Could not resolve type in @extends tag of YourType");
}
public void testForwardTypeDeclaration6() throws Exception {
testClosureTypesMultipleWarnings(
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/**\n" +
" * @constructor\n" +
" * @implements {MyType}\n" +
" */ var YourType = function() {};" +
"/** @override */ YourType.prototype.method = function() {};",
Lists.newArrayList(
"Could not resolve type in @implements tag of YourType",
"property method not defined on any superclass of YourType"));
}
public void testForwardTypeDeclaration7() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/** @param {MyType=} x */" +
"function f(x) { return x == undefined; }", null);
}
public void testForwardTypeDeclaration8() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/** @param {MyType} x */" +
"function f(x) { return x.name == undefined; }", null);
}
public void testForwardTypeDeclaration9() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/** @param {MyType} x */" +
"function f(x) { x.name = 'Bob'; }", null);
}
public void testForwardTypeDeclaration10() throws Exception {
String f = "goog.addDependency('zzz.js', ['MyType'], []);" +
"/** @param {MyType|number} x */ function f(x) { }";
testClosureTypes(f, null);
testClosureTypes(f + "f(3);", null);
testClosureTypes(f + "f('3');",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: (MyType|null|number)");
}
public void testDuplicateTypeDef() throws Exception {
testTypes(
"var goog = {};" +
"/** @constructor */ goog.Bar = function() {};" +
"/** @typedef {number} */ goog.Bar;",
"variable goog.Bar redefined with type None, " +
"original definition at [testcode]:1 " +
"with type function (new:goog.Bar): undefined");
}
public void testTypeDef1() throws Exception {
testTypes(
"var goog = {};" +
"/** @typedef {number} */ goog.Bar;" +
"/** @param {goog.Bar} x */ function f(x) {}" +
"f(3);");
}
public void testTypeDef2() throws Exception {
testTypes(
"var goog = {};" +
"/** @typedef {number} */ goog.Bar;" +
"/** @param {goog.Bar} x */ function f(x) {}" +
"f('3');",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testTypeDef3() throws Exception {
testTypes(
"var goog = {};" +
"/** @typedef {number} */ var Bar;" +
"/** @param {Bar} x */ function f(x) {}" +
"f('3');",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testTypeDef4() throws Exception {
testTypes(
"/** @constructor */ function A() {}" +
"/** @constructor */ function B() {}" +
"/** @typedef {(A|B)} */ var AB;" +
"/** @param {AB} x */ function f(x) {}" +
"f(new A()); f(new B()); f(1);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: (A|B|null)");
}
public void testTypeDef5() throws Exception {
// Notice that the error message is slightly different than
// the one for testTypeDef4, even though they should be the same.
// This is an implementation detail necessary for NamedTypes work out
// ok, and it should change if NamedTypes ever go away.
testTypes(
"/** @param {AB} x */ function f(x) {}" +
"/** @constructor */ function A() {}" +
"/** @constructor */ function B() {}" +
"/** @typedef {(A|B)} */ var AB;" +
"f(new A()); f(new B()); f(1);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: (A|B|null)");
}
public void testCircularTypeDef() throws Exception {
testTypes(
"var goog = {};" +
"/** @typedef {number|Array.<goog.Bar>} */ goog.Bar;" +
"/** @param {goog.Bar} x */ function f(x) {}" +
"f(3); f([3]); f([[3]]);");
}
public void testGetTypedPercent1() throws Exception {
String js = "var id = function(x) { return x; }\n" +
"var id2 = function(x) { return id(x); }";
assertEquals(50.0, getTypedPercent(js), 0.1);
}
public void testGetTypedPercent2() throws Exception {
String js = "var x = {}; x.y = 1;";
assertEquals(100.0, getTypedPercent(js), 0.1);
}
public void testGetTypedPercent3() throws Exception {
String js = "var f = function(x) { x.a = x.b; }";
assertEquals(50.0, getTypedPercent(js), 0.1);
}
public void testGetTypedPercent4() throws Exception {
String js = "var n = {};\n /** @constructor */ n.T = function() {};\n" +
"/** @type n.T */ var x = new n.T();";
assertEquals(100.0, getTypedPercent(js), 0.1);
}
public void testGetTypedPercent5() throws Exception {
String js = "/** @enum {number} */ keys = {A: 1,B: 2,C: 3};";
assertEquals(100.0, getTypedPercent(js), 0.1);
}
public void testGetTypedPercent6() throws Exception {
String js = "a = {TRUE: 1, FALSE: 0};";
assertEquals(100.0, getTypedPercent(js), 0.1);
}
private double getTypedPercent(String js) throws Exception {
Node n = compiler.parseTestCode(js);
Node externs = new Node(Token.BLOCK);
Node externAndJsRoot = new Node(Token.BLOCK, externs, n);
externAndJsRoot.setIsSyntheticBlock(true);
TypeCheck t = makeTypeCheck();
t.processForTesting(null, n);
return t.getTypedPercent();
}
private ObjectType getInstanceType(Node js1Node) {
JSType type = js1Node.getFirstChild().getJSType();
assertNotNull(type);
assertTrue(type instanceof FunctionType);
FunctionType functionType = (FunctionType) type;
assertTrue(functionType.isConstructor());
return functionType.getInstanceType();
}
public void testPrototypePropertyReference() throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope(""
+ "/** @constructor */\n"
+ "function Foo() {}\n"
+ "/** @param {number} a */\n"
+ "Foo.prototype.bar = function(a){};\n"
+ "/** @param {Foo} f */\n"
+ "function baz(f) {\n"
+ " Foo.prototype.bar.call(f, 3);\n"
+ "}");
assertEquals(0, compiler.getErrorCount());
assertEquals(0, compiler.getWarningCount());
assertTrue(p.scope.getVar("Foo").getType() instanceof FunctionType);
FunctionType fooType = (FunctionType) p.scope.getVar("Foo").getType();
assertEquals("function (this:Foo, number): undefined",
fooType.getPrototype().getPropertyType("bar").toString());
}
public void testResolvingNamedTypes() throws Exception {
String js = ""
+ "/** @constructor */\n"
+ "var Foo = function() {}\n"
+ "/** @param {number} a */\n"
+ "Foo.prototype.foo = function(a) {\n"
+ " return this.baz().toString();\n"
+ "};\n"
+ "/** @return {Baz} */\n"
+ "Foo.prototype.baz = function() { return new Baz(); };\n"
+ "/** @constructor\n"
+ " * @extends Foo */\n"
+ "var Bar = function() {};"
+ "/** @constructor */\n"
+ "var Baz = function() {};";
assertEquals(100.0, getTypedPercent(js), 0.1);
}
public void testMissingProperty1() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() { return this.a; };" +
"Foo.prototype.baz = function() { this.a = 3; };");
}
public void testMissingProperty2() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() { return this.a; };" +
"Foo.prototype.baz = function() { this.b = 3; };",
"Property a never defined on Foo");
}
public void testMissingProperty3() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() { return this.a; };" +
"(new Foo).a = 3;");
}
public void testMissingProperty4() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() { return this.a; };" +
"(new Foo).b = 3;",
"Property a never defined on Foo");
}
public void testMissingProperty5() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() { return this.a; };" +
"/** @constructor */ function Bar() { this.a = 3; };",
"Property a never defined on Foo");
}
public void testMissingProperty6() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() { return this.a; };" +
"/** @constructor \n * @extends {Foo} */ " +
"function Bar() { this.a = 3; };");
}
public void testMissingProperty7() throws Exception {
testTypes(
"/** @param {Object} obj */" +
"function foo(obj) { return obj.impossible; }",
"Property impossible never defined on Object");
}
public void testMissingProperty8() throws Exception {
testTypes(
"/** @param {Object} obj */" +
"function foo(obj) { return typeof obj.impossible; }");
}
public void testMissingProperty9() throws Exception {
testTypes(
"/** @param {Object} obj */" +
"function foo(obj) { if (obj.impossible) { return true; } }");
}
public void testMissingProperty10() throws Exception {
testTypes(
"/** @param {Object} obj */" +
"function foo(obj) { while (obj.impossible) { return true; } }");
}
public void testMissingProperty11() throws Exception {
testTypes(
"/** @param {Object} obj */" +
"function foo(obj) { for (;obj.impossible;) { return true; } }");
}
public void testMissingProperty12() throws Exception {
testTypes(
"/** @param {Object} obj */" +
"function foo(obj) { do { } while (obj.impossible); }");
}
public void testMissingProperty13() throws Exception {
testTypes(
"var goog = {}; goog.isDef = function(x) { return false; };" +
"/** @param {Object} obj */" +
"function foo(obj) { return goog.isDef(obj.impossible); }");
}
public void testMissingProperty14() throws Exception {
testTypes(
"var goog = {}; goog.isDef = function(x) { return false; };" +
"/** @param {Object} obj */" +
"function foo(obj) { return goog.isNull(obj.impossible); }",
"Property isNull never defined on goog");
}
public void testMissingProperty15() throws Exception {
testTypes(
"/** @param {Object} x */" +
"function f(x) { if (x.foo) { x.foo(); } }");
}
public void testMissingProperty16() throws Exception {
testTypes(
"/** @param {Object} x */" +
"function f(x) { x.foo(); if (x.foo) {} }",
"Property foo never defined on Object");
}
public void testMissingProperty17() throws Exception {
testTypes(
"/** @param {Object} x */" +
"function f(x) { if (typeof x.foo == 'function') { x.foo(); } }");
}
public void testMissingProperty18() throws Exception {
testTypes(
"/** @param {Object} x */" +
"function f(x) { if (x.foo instanceof Function) { x.foo(); } }");
}
public void testMissingProperty19() throws Exception {
testTypes(
"/** @param {Object} x */" +
"function f(x) { if (x.bar) { if (x.foo) {} } else { x.foo(); } }",
"Property foo never defined on Object");
}
public void testMissingProperty20() throws Exception {
// NOTE(nicksantos): In the else branch, we know that x.foo is a
// CHECKED_UNKNOWN (UNKNOWN restricted to a falsey value). We could
// do some more sophisticated analysis here. Obviously, if x.foo is false,
// then x.foo cannot possibly be called. For example, you could imagine a
// VagueType that was like UnknownType, but had some constraints on it
// so that we knew it could never be a function.
//
// For now, we just punt on this issue.
testTypes(
"/** @param {Object} x */" +
"function f(x) { if (x.foo) { } else { x.foo(); } }");
}
public void testMissingProperty21() throws Exception {
testTypes(
"/** @param {Object} x */" +
"function f(x) { x.foo && x.foo(); }");
}
public void testMissingProperty22() throws Exception {
testTypes(
"/** @param {Object} x \n * @return {boolean} */" +
"function f(x) { return x.foo ? x.foo() : true; }");
}
public void testMissingProperty23() throws Exception {
testTypes(
"function f(x) { x.impossible(); }",
"Property impossible never defined on x");
}
public void testMissingProperty24() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MissingType'], []);" +
"/** @param {MissingType} x */" +
"function f(x) { x.impossible(); }", null);
}
public void testMissingProperty25() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {};" +
"Foo.prototype.bar = function() {};" +
"/** @constructor */ var FooAlias = Foo;" +
"(new FooAlias()).bar();");
}
public void testMissingProperty26() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {};" +
"/** @constructor */ var FooAlias = Foo;" +
"FooAlias.prototype.bar = function() {};" +
"(new Foo()).bar();");
}
public void testMissingProperty27() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MissingType'], []);" +
"/** @param {?MissingType} x */" +
"function f(x) {" +
" for (var parent = x; parent; parent = parent.getParent()) {}" +
"}", null);
}
public void testMissingProperty28() throws Exception {
testTypes(
"function f(obj) {" +
" /** @type {*} */ obj.foo;" +
" return obj.foo;" +
"}");
testTypes(
"function f(obj) {" +
" /** @type {*} */ obj.foo;" +
" return obj.foox;" +
"}",
"Property foox never defined on obj");
}
public void testMissingProperty29() throws Exception {
// This used to emit a warning.
testTypes(
// externs
"/** @constructor */ var Foo;" +
"Foo.prototype.opera;" +
"Foo.prototype.opera.postError;",
"",
null,
false);
}
public void testMissingProperty30() throws Exception {
testTypes(
"/** @return {*} */" +
"function f() {" +
" return {};" +
"}" +
"f().a = 3;" +
"/** @param {Object} y */ function g(y) { return y.a; }");
}
public void testMissingProperty31() throws Exception {
testTypes(
"/** @return {Array|number} */" +
"function f() {" +
" return [];" +
"}" +
"f().a = 3;" +
"/** @param {Array} y */ function g(y) { return y.a; }");
}
public void testMissingProperty32() throws Exception {
testTypes(
"/** @return {Array|number} */" +
"function f() {" +
" return [];" +
"}" +
"f().a = 3;" +
"/** @param {Date} y */ function g(y) { return y.a; }",
"Property a never defined on Date");
}
public void testMissingProperty33() throws Exception {
testTypes(
"/** @param {Object} x */" +
"function f(x) { !x.foo || x.foo(); }");
}
public void testMissingProperty34() throws Exception {
testTypes(
"/** @fileoverview \n * @suppress {missingProperties} */" +
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() { return this.a; };" +
"Foo.prototype.baz = function() { this.b = 3; };");
}
public void testMissingProperty35() throws Exception {
// Bar has specialProp defined, so Bar|Baz may have specialProp defined.
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @constructor */ function Bar() {}" +
"/** @constructor */ function Baz() {}" +
"/** @param {Foo|Bar} x */ function f(x) { x.specialProp = 1; }" +
"/** @param {Bar|Baz} x */ function g(x) { return x.specialProp; }");
}
public void testMissingProperty36() throws Exception {
// Foo has baz defined, and SubFoo has bar defined, so some objects with
// bar may have baz.
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.baz = 0;" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"SubFoo.prototype.bar = 0;" +
"/** @param {{bar: number}} x */ function f(x) { return x.baz; }");
}
public void testMissingProperty37() throws Exception {
// This used to emit a missing property warning because we couldn't
// determine that the inf(Foo, {isVisible:boolean}) == SubFoo.
testTypes(
"/** @param {{isVisible: boolean}} x */ function f(x){" +
" x.isVisible = false;" +
"}" +
"/** @constructor */ function Foo() {}" +
"/**\n" +
" * @constructor \n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/** @type {boolean} */ SubFoo.prototype.isVisible = true;" +
"/**\n" +
" * @param {Foo} x\n" +
" * @return {boolean}\n" +
" */\n" +
"function g(x) { return x.isVisible; }");
}
public void testMissingProperty38() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @constructor */ function Bar() {}" +
"/** @return {Foo|Bar} */ function f() { return new Foo(); }" +
"f().missing;",
"Property missing never defined on (Bar|Foo|null)");
}
public void testMissingProperty39() throws Exception {
testTypes(
"/** @return {string|number} */ function f() { return 3; }" +
"f().length;");
}
public void testMissingProperty40() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MissingType'], []);" +
"/** @param {(Array|MissingType)} x */" +
"function f(x) { x.impossible(); }", null);
}
public void testMissingProperty41() throws Exception {
testTypes(
"/** @param {(Array|Date)} x */" +
"function f(x) { if (x.impossible) x.impossible(); }");
}
public void testReflectObject1() throws Exception {
testClosureTypes(
"var goog = {}; goog.reflect = {}; " +
"goog.reflect.object = function(x, y){};" +
"/** @constructor */ function A() {}" +
"goog.reflect.object(A, {x: 3});",
null);
}
public void testReflectObject2() throws Exception {
testClosureTypes(
"var goog = {}; goog.reflect = {}; " +
"goog.reflect.object = function(x, y){};" +
"/** @param {string} x */ function f(x) {}" +
"/** @constructor */ function A() {}" +
"goog.reflect.object(A, {x: f(1 + 1)});",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testLends1() throws Exception {
testTypes(
"function extend(x, y) {}" +
"/** @constructor */ function Foo() {}" +
"extend(Foo, /** @lends */ ({bar: 1}));",
"Bad type annotation. missing object name in @lends tag");
}
public void testLends2() throws Exception {
testTypes(
"function extend(x, y) {}" +
"/** @constructor */ function Foo() {}" +
"extend(Foo, /** @lends {Foob} */ ({bar: 1}));",
"Variable Foob not declared before @lends annotation.");
}
public void testLends3() throws Exception {
testTypes(
"function extend(x, y) {}" +
"/** @constructor */ function Foo() {}" +
"extend(Foo, {bar: 1});" +
"alert(Foo.bar);",
"Property bar never defined on Foo");
}
public void testLends4() throws Exception {
testTypes(
"function extend(x, y) {}" +
"/** @constructor */ function Foo() {}" +
"extend(Foo, /** @lends {Foo} */ ({bar: 1}));" +
"alert(Foo.bar);");
}
public void testLends5() throws Exception {
testTypes(
"function extend(x, y) {}" +
"/** @constructor */ function Foo() {}" +
"extend(Foo, {bar: 1});" +
"alert((new Foo()).bar);",
"Property bar never defined on Foo");
}
public void testLends6() throws Exception {
testTypes(
"function extend(x, y) {}" +
"/** @constructor */ function Foo() {}" +
"extend(Foo, /** @lends {Foo.prototype} */ ({bar: 1}));" +
"alert((new Foo()).bar);");
}
public void testLends7() throws Exception {
testTypes(
"function extend(x, y) {}" +
"/** @constructor */ function Foo() {}" +
"extend(Foo, /** @lends {Foo.prototype|Foo} */ ({bar: 1}));",
"Bad type annotation. expected closing }");
}
public void testLends8() throws Exception {
testTypes(
"function extend(x, y) {}" +
"/** @type {number} */ var Foo = 3;" +
"extend(Foo, /** @lends {Foo} */ ({bar: 1}));",
"May only lend properties to object types. Foo has type number.");
}
public void testLends9() throws Exception {
testClosureTypesMultipleWarnings(
"function extend(x, y) {}" +
"/** @constructor */ function Foo() {}" +
"extend(Foo, /** @lends {!Foo} */ ({bar: 1}));",
Lists.newArrayList(
"Bad type annotation. expected closing }",
"Bad type annotation. missing object name in @lends tag"));
}
public void testLends10() throws Exception {
testTypes(
"function defineClass(x) { return function() {}; } " +
"/** @constructor */" +
"var Foo = defineClass(" +
" /** @lends {Foo.prototype} */ ({/** @type {number} */ bar: 1}));" +
"/** @return {string} */ function f() { return (new Foo()).bar; }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testLends11() throws Exception {
testTypes(
"function defineClass(x, y) { return function() {}; } " +
"/** @constructor */" +
"var Foo = function() {};" +
"/** @return {*} */ Foo.prototype.bar = function() { return 3; };" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */\n" +
"var SubFoo = defineClass(Foo, " +
" /** @lends {SubFoo.prototype} */ ({\n" +
" /** @return {number} */ bar: function() { return 3; }}));" +
"/** @return {string} */ function f() { return (new SubFoo()).bar(); }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testDeclaredNativeTypeEquality() throws Exception {
Node n = parseAndTypeCheck("/** @constructor */ function Object() {};");
assertEquals(registry.getNativeType(JSTypeNative.OBJECT_FUNCTION_TYPE),
n.getFirstChild().getJSType());
}
public void testUndefinedVar() throws Exception {
Node n = parseAndTypeCheck("var undefined;");
assertEquals(registry.getNativeType(JSTypeNative.VOID_TYPE),
n.getFirstChild().getFirstChild().getJSType());
}
public void testFlowScopeBug1() throws Exception {
Node n = parseAndTypeCheck("/** @param {number} a \n"
+ "* @param {number} b */\n"
+ "function f(a, b) {\n"
+ "/** @type number */"
+ "var i = 0;"
+ "for (; (i + a) < b; ++i) {}}");
// check the type of the add node for i + f
assertEquals(registry.getNativeType(JSTypeNative.NUMBER_TYPE),
n.getFirstChild().getLastChild().getLastChild().getFirstChild()
.getNext().getFirstChild().getJSType());
}
public void testFlowScopeBug2() throws Exception {
Node n = parseAndTypeCheck("/** @constructor */ function Foo() {};\n"
+ "Foo.prototype.hi = false;"
+ "function foo(a, b) {\n"
+ " /** @type Array */"
+ " var arr;"
+ " /** @type number */"
+ " var iter;"
+ " for (iter = 0; iter < arr.length; ++ iter) {"
+ " /** @type Foo */"
+ " var afoo = arr[iter];"
+ " afoo;"
+ " }"
+ "}");
// check the type of afoo when referenced
assertEquals(registry.createNullableType(registry.getType("Foo")),
n.getLastChild().getLastChild().getLastChild().getLastChild()
.getLastChild().getLastChild().getJSType());
}
public void testAddSingletonGetter() {
Node n = parseAndTypeCheck(
"/** @constructor */ function Foo() {};\n" +
"goog.addSingletonGetter(Foo);");
ObjectType o = (ObjectType) n.getFirstChild().getJSType();
assertEquals("function (): Foo",
o.getPropertyType("getInstance").toString());
assertEquals("Foo", o.getPropertyType("instance_").toString());
}
public void testTypeCheckStandaloneAST() throws Exception {
Node n = compiler.parseTestCode("function Foo() { }");
typeCheck(n);
TypedScopeCreator scopeCreator = new TypedScopeCreator(compiler);
Scope topScope = scopeCreator.createScope(n, null);
Node second = compiler.parseTestCode("new Foo");
Node externs = new Node(Token.BLOCK);
Node externAndJsRoot = new Node(Token.BLOCK, externs, second);
externAndJsRoot.setIsSyntheticBlock(true);
new TypeCheck(
compiler,
new SemanticReverseAbstractInterpreter(
compiler.getCodingConvention(), registry),
registry, topScope, scopeCreator, CheckLevel.WARNING, CheckLevel.OFF)
.process(null, second);
assertEquals(1, compiler.getWarningCount());
assertEquals("cannot instantiate non-constructor",
compiler.getWarnings()[0].description);
}
public void testUpdateParameterTypeOnClosure() throws Exception {
testTypes(
"/**\n" +
"* @constructor\n" +
"* @param {*=} opt_value\n" +
"* @return {?}\n" +
"*/\n" +
"function Object(opt_value) {}\n" +
"/**\n" +
"* @constructor\n" +
"* @param {...*} var_args\n" +
"*/\n" +
"function Function(var_args) {}\n" +
"/**\n" +
"* @type {Function}\n" +
"*/\n" +
// The line below sets JSDocInfo on Object so that the type of the
// argument to function f has JSDoc through its prototype chain.
"Object.prototype.constructor = function() {};\n",
"/**\n" +
"* @param {function(): boolean} fn\n" +
"*/\n" +
"function f(fn) {}\n" +
"f(function(g) { });\n",
null,
false);
}
public void testBadTemplateType1() throws Exception {
testTypes(
"/**\n" +
"* @param {T} x\n" +
"* @param {T} y\n" +
"* @param {function(this:T, ...)} z\n" +
"* @template T\n" +
"*/\n" +
"function f(x, y, z) {}\n" +
"f(this, this, function() { this });",
FunctionTypeBuilder.TEMPLATE_TYPE_DUPLICATED.format());
}
public void testBadTemplateType2() throws Exception {
testTypes(
"/**\n" +
"* @param {T} x\n" +
"* @param {function(this:T, ...)} y\n" +
"* @template T\n" +
"*/\n" +
"function f(x, y) {}\n" +
"f(0, function() {});",
TypeInference.TEMPLATE_TYPE_NOT_OBJECT_TYPE.format("number"));
}
public void testBadTemplateType3() throws Exception {
testTypes(
"/**\n" +
" * @param {T} x\n" +
" * @template T\n" +
"*/\n" +
"function f(x) {}\n" +
"f(this);",
TypeInference.TEMPLATE_TYPE_OF_THIS_EXPECTED.format());
}
public void testBadTemplateType4() throws Exception {
testTypes(
"/**\n" +
"* @template T\n" +
"*/\n" +
"function f() {}\n" +
"f();",
FunctionTypeBuilder.TEMPLATE_TYPE_EXPECTED.format());
}
public void testBadTemplateType5() throws Exception {
testTypes(
"/**\n" +
"* @template T\n" +
"* @return {T}\n" +
"*/\n" +
"function f() {}\n" +
"f();",
FunctionTypeBuilder.TEMPLATE_TYPE_EXPECTED.format());
}
public void testFunctionLiteralUndefinedThisArgument() throws Exception {
testTypes(""
+ "/**\n"
+ " * @param {function(this:T, ...)?} fn\n"
+ " * @param {?T} opt_obj\n"
+ " * @template T\n"
+ " */\n"
+ "function baz(fn, opt_obj) {}\n"
+ "baz(function() { this; });",
"Function literal argument refers to undefined this argument");
}
public void testFunctionLiteralDefinedThisArgument() throws Exception {
testTypes(""
+ "/**\n"
+ " * @param {function(this:T, ...)?} fn\n"
+ " * @param {?T} opt_obj\n"
+ " * @template T\n"
+ " */\n"
+ "function baz(fn, opt_obj) {}\n"
+ "baz(function() { this; }, {});");
}
public void testFunctionLiteralUnreadNullThisArgument() throws Exception {
testTypes(""
+ "/**\n"
+ " * @param {function(this:T, ...)?} fn\n"
+ " * @param {?T} opt_obj\n"
+ " * @template T\n"
+ " */\n"
+ "function baz(fn, opt_obj) {}\n"
+ "baz(function() {}, null);");
}
public void testUnionTemplateThisType() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** @return {F|Array} */ function g() { return []; }" +
"/** @param {F} x */ function h(x) { }" +
"/**\n" +
"* @param {T} x\n" +
"* @param {function(this:T, ...)} y\n" +
"* @template T\n" +
"*/\n" +
"function f(x, y) {}\n" +
"f(g(), function() { h(this); });",
"actual parameter 1 of h does not match formal parameter\n" +
"found : Object\n" +
"required: (F|null)");
}
public void testActiveXObject() throws Exception {
testTypes(
"/** @type {Object} */ var x = new ActiveXObject();" +
"/** @type { {impossibleProperty} } */ var y = new ActiveXObject();");
}
public void testRecordType1() throws Exception {
testTypes(
"/** @param {{prop: number}} x */" +
"function f(x) {}" +
"f({});",
"actual parameter 1 of f does not match formal parameter\n" +
"found : {prop: (number|undefined)}\n" +
"required: {prop: number}");
}
public void testRecordType2() throws Exception {
testTypes(
"/** @param {{prop: (number|undefined)}} x */" +
"function f(x) {}" +
"f({});");
}
public void testRecordType3() throws Exception {
testTypes(
"/** @param {{prop: number}} x */" +
"function f(x) {}" +
"f({prop: 'x'});",
"actual parameter 1 of f does not match formal parameter\n" +
"found : {prop: (number|string)}\n" +
"required: {prop: number}");
}
public void testRecordType4() throws Exception {
// Notice that we do not do flow-based inference on the object type:
// We don't try to prove that x.prop may not be string until x
// gets passed to g.
testClosureTypesMultipleWarnings(
"/** @param {{prop: (number|undefined)}} x */" +
"function f(x) {}" +
"/** @param {{prop: (string|undefined)}} x */" +
"function g(x) {}" +
"var x = {}; f(x); g(x);",
Lists.newArrayList(
"actual parameter 1 of f does not match formal parameter\n" +
"found : {prop: (number|string|undefined)}\n" +
"required: {prop: (number|undefined)}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : {prop: (number|string|undefined)}\n" +
"required: {prop: (string|undefined)}"));
}
public void testRecordType5() throws Exception {
testTypes(
"/** @param {{prop: (number|undefined)}} x */" +
"function f(x) {}" +
"/** @param {{otherProp: (string|undefined)}} x */" +
"function g(x) {}" +
"var x = {}; f(x); g(x);");
}
public void testRecordType6() throws Exception {
testTypes(
"/** @return {{prop: (number|undefined)}} x */" +
"function f() { return {}; }");
}
public void testRecordType7() throws Exception {
testTypes(
"/** @return {{prop: (number|undefined)}} x */" +
"function f() { var x = {}; g(x); return x; }" +
"/** @param {number} x */" +
"function g(x) {}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : {prop: (number|undefined)}\n" +
"required: number");
}
public void testRecordType8() throws Exception {
testTypes(
"/** @return {{prop: (number|string)}} x */" +
"function f() { var x = {prop: 3}; g(x.prop); return x; }" +
"/** @param {string} x */" +
"function g(x) {}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testDuplicateRecordFields1() throws Exception {
testTypes("/**"
+ "* @param {{x:string, x:number}} a"
+ "*/"
+ "function f(a) {};",
"Parse error. Duplicate record field x");
}
public void testDuplicateRecordFields2() throws Exception {
testTypes("/**"
+ "* @param {{name:string,number:x,number:y}} a"
+ " */"
+ "function f(a) {};",
new String[] {"Bad type annotation. Unknown type x",
"Parse error. Duplicate record field number",
"Bad type annotation. Unknown type y"});
}
public void testMultipleExtendsInterface1() throws Exception {
testTypes("/** @interface */ function base1() {}\n"
+ "/** @interface */ function base2() {}\n"
+ "/** @interface\n"
+ "* @extends {base1}\n"
+ "* @extends {base2}\n"
+ "*/\n"
+ "function derived() {}");
}
public void testMultipleExtendsInterface2() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @desc description */Int0.prototype.foo = function() {};" +
"/** @interface \n @extends {Int0} \n @extends {Int1} */" +
"function Int2() {};" +
"/** @constructor\n @implements {Int2} */function Foo() {};",
"property foo on interface Int0 is not implemented by type Foo");
}
public void testMultipleExtendsInterface3() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @desc description */Int1.prototype.foo = function() {};" +
"/** @interface \n @extends {Int0} \n @extends {Int1} */" +
"function Int2() {};" +
"/** @constructor\n @implements {Int2} */function Foo() {};",
"property foo on interface Int1 is not implemented by type Foo");
}
public void testMultipleExtendsInterface4() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @interface \n @extends {Int0} \n @extends {Int1} \n" +
" @extends {number} */" +
"function Int2() {};" +
"/** @constructor\n @implements {Int2} */function Foo() {};",
"Int2 @extends non-object type number");
}
public void testMultipleExtendsInterface5() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @constructor */function Int1() {};" +
"/** @desc description @ return {string} x */" +
"/** @interface \n @extends {Int0} \n @extends {Int1} */" +
"function Int2() {};",
"Int2 cannot extend this type; a constructor can only extend " +
"objects and an interface can only extend interfaces");
}
public void testMultipleExtendsInterface6() throws Exception {
testTypes(
"/** @interface */function Super1() {};" +
"/** @interface */function Super2() {};" +
"/** @param {number} bar */Super2.prototype.foo = function(bar) {};" +
"/** @interface\n @extends {Super1}\n " +
"@extends {Super2} */function Sub() {};" +
"/** @override\n @param {string} bar */Sub.prototype.foo =\n" +
"function(bar) {};",
"mismatch of the foo property type and the type of the property it " +
"overrides from superclass Super2\n" +
"original: function (this:Super2, number): undefined\n" +
"override: function (this:Sub, string): undefined");
}
public void testMultipleExtendsInterfaceAssignment() throws Exception {
testTypes("/** @interface */var I1 = function() {};\n" +
"/** @interface */ var I2 = function() {}\n" +
"/** @interface\n@extends {I1}\n@extends {I2}*/" +
"var I3 = function() {};\n" +
"/** @constructor\n@implements {I3}*/var T = function() {};\n" +
"var t = new T();\n" +
"/** @type {I1} */var i1 = t;\n" +
"/** @type {I2} */var i2 = t;\n" +
"/** @type {I3} */var i3 = t;\n" +
"i1 = i3;\n" +
"i2 = i3;\n");
}
public void testMultipleExtendsInterfaceParamPass() throws Exception {
testTypes("/** @interface */var I1 = function() {};\n" +
"/** @interface */ var I2 = function() {}\n" +
"/** @interface\n@extends {I1}\n@extends {I2}*/" +
"var I3 = function() {};\n" +
"/** @constructor\n@implements {I3}*/var T = function() {};\n" +
"var t = new T();\n" +
"/** @param x I1 \n@param y I2\n@param z I3*/function foo(x,y,z){};\n" +
"foo(t,t,t)\n");
}
public void testBadMultipleExtendsClass() throws Exception {
testTypes("/** @constructor */ function base1() {}\n"
+ "/** @constructor */ function base2() {}\n"
+ "/** @constructor\n"
+ "* @extends {base1}\n"
+ "* @extends {base2}\n"
+ "*/\n"
+ "function derived() {}",
"Bad type annotation. type annotation incompatible "
+ "with other annotations");
}
public void testInterfaceExtendsResolution() throws Exception {
testTypes("/** @interface \n @extends {A} */ function B() {};\n" +
"/** @constructor \n @implements {B} */ function C() {};\n" +
"/** @interface */ function A() {};");
}
public void testPropertyCanBeDefinedInObject() throws Exception {
testTypes("/** @interface */ function I() {};" +
"I.prototype.bar = function() {};" +
"/** @type {Object} */ var foo;" +
"foo.bar();");
}
private void checkObjectType(ObjectType objectType, String propertyName,
JSType expectedType) {
assertTrue("Expected " + objectType.getReferenceName() +
" to have property " +
propertyName, objectType.hasProperty(propertyName));
assertEquals("Expected " + objectType.getReferenceName() +
"'s property " +
propertyName + " to have type " + expectedType,
expectedType, objectType.getPropertyType(propertyName));
}
public void testExtendedInterfacePropertiesCompatibility1() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @type {number} */" +
"Int0.prototype.foo;" +
"/** @type {string} */" +
"Int1.prototype.foo;" +
"/** @interface \n @extends {Int0} \n @extends {Int1} */" +
"function Int2() {};",
"Interface Int2 has a property foo with incompatible types in its " +
"super interfaces Int0 and Int1");
}
public void testExtendedInterfacePropertiesCompatibility2() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @interface */function Int2() {};" +
"/** @type {number} */" +
"Int0.prototype.foo;" +
"/** @type {string} */" +
"Int1.prototype.foo;" +
"/** @type {Object} */" +
"Int2.prototype.foo;" +
"/** @interface \n @extends {Int0} \n @extends {Int1} \n" +
"@extends {Int2}*/" +
"function Int3() {};",
new String[] {
"Interface Int3 has a property foo with incompatible types in " +
"its super interfaces Int0 and Int1",
"Interface Int3 has a property foo with incompatible types in " +
"its super interfaces Int1 and Int2"
});
}
public void testExtendedInterfacePropertiesCompatibility3() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @type {number} */" +
"Int0.prototype.foo;" +
"/** @type {string} */" +
"Int1.prototype.foo;" +
"/** @interface \n @extends {Int1} */ function Int2() {};" +
"/** @interface \n @extends {Int0} \n @extends {Int2} */" +
"function Int3() {};",
"Interface Int3 has a property foo with incompatible types in its " +
"super interfaces Int0 and Int1");
}
public void testExtendedInterfacePropertiesCompatibility4() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface \n @extends {Int0} */ function Int1() {};" +
"/** @type {number} */" +
"Int0.prototype.foo;" +
"/** @interface */function Int2() {};" +
"/** @interface \n @extends {Int2} */ function Int3() {};" +
"/** @type {string} */" +
"Int2.prototype.foo;" +
"/** @interface \n @extends {Int1} \n @extends {Int3} */" +
"function Int4() {};",
"Interface Int4 has a property foo with incompatible types in its " +
"super interfaces Int0 and Int2");
}
public void testExtendedInterfacePropertiesCompatibility5() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @type {number} */" +
"Int0.prototype.foo;" +
"/** @type {string} */" +
"Int1.prototype.foo;" +
"/** @interface \n @extends {Int1} */ function Int2() {};" +
"/** @interface \n @extends {Int0} \n @extends {Int2} */" +
"function Int3() {};" +
"/** @interface */function Int4() {};" +
"/** @type {number} */" +
"Int4.prototype.foo;" +
"/** @interface \n @extends {Int3} \n @extends {Int4} */" +
"function Int5() {};",
new String[] {
"Interface Int3 has a property foo with incompatible types in its" +
" super interfaces Int0 and Int1",
"Interface Int5 has a property foo with incompatible types in its" +
" super interfaces Int1 and Int4"});
}
public void testExtendedInterfacePropertiesCompatibility6() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @type {number} */" +
"Int0.prototype.foo;" +
"/** @type {string} */" +
"Int1.prototype.foo;" +
"/** @interface \n @extends {Int1} */ function Int2() {};" +
"/** @interface \n @extends {Int0} \n @extends {Int2} */" +
"function Int3() {};" +
"/** @interface */function Int4() {};" +
"/** @type {string} */" +
"Int4.prototype.foo;" +
"/** @interface \n @extends {Int3} \n @extends {Int4} */" +
"function Int5() {};",
"Interface Int3 has a property foo with incompatible types in its" +
" super interfaces Int0 and Int1");
}
public void testExtendedInterfacePropertiesCompatibility7() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @type {number} */" +
"Int0.prototype.foo;" +
"/** @type {string} */" +
"Int1.prototype.foo;" +
"/** @interface \n @extends {Int1} */ function Int2() {};" +
"/** @interface \n @extends {Int0} \n @extends {Int2} */" +
"function Int3() {};" +
"/** @interface */function Int4() {};" +
"/** @type {Object} */" +
"Int4.prototype.foo;" +
"/** @interface \n @extends {Int3} \n @extends {Int4} */" +
"function Int5() {};",
new String[] {
"Interface Int3 has a property foo with incompatible types in its" +
" super interfaces Int0 and Int1",
"Interface Int5 has a property foo with incompatible types in its" +
" super interfaces Int1 and Int4"});
}
public void testExtendedInterfacePropertiesCompatibility8() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @type {number} */" +
"Int0.prototype.foo;" +
"/** @type {string} */" +
"Int1.prototype.bar;" +
"/** @interface \n @extends {Int1} */ function Int2() {};" +
"/** @interface \n @extends {Int0} \n @extends {Int2} */" +
"function Int3() {};" +
"/** @interface */function Int4() {};" +
"/** @type {Object} */" +
"Int4.prototype.foo;" +
"/** @type {Null} */" +
"Int4.prototype.bar;" +
"/** @interface \n @extends {Int3} \n @extends {Int4} */" +
"function Int5() {};",
new String[] {
"Interface Int5 has a property bar with incompatible types in its" +
" super interfaces Int1 and Int4",
"Interface Int5 has a property foo with incompatible types in its" +
" super interfaces Int0 and Int4"});
}
private void testTypes(String js) throws Exception {
testTypes(js, (String) null);
}
private void testTypes(String js, String description) throws Exception {
testTypes(js, description, false);
}
private void testTypes(String js, DiagnosticType type) throws Exception {
testTypes(js, type.format(), false);
}
private void testClosureTypes(String js, String description)
throws Exception {
testClosureTypesMultipleWarnings(js,
description == null ? null : Lists.newArrayList(description));
}
private void testClosureTypesMultipleWarnings(
String js, List<String> descriptions) throws Exception {
Node n = compiler.parseTestCode(js);
Node externs = new Node(Token.BLOCK);
Node externAndJsRoot = new Node(Token.BLOCK, externs, n);
externAndJsRoot.setIsSyntheticBlock(true);
assertEquals("parsing error: " +
Joiner.on(", ").join(compiler.getErrors()),
0, compiler.getErrorCount());
// For processing goog.addDependency for forward typedefs.
new ProcessClosurePrimitives(compiler, null, CheckLevel.ERROR, true)
.process(null, n);
CodingConvention convention = compiler.getCodingConvention();
new TypeCheck(compiler,
new ClosureReverseAbstractInterpreter(
convention, registry).append(
new SemanticReverseAbstractInterpreter(
convention, registry))
.getFirst(),
registry)
.processForTesting(null, n);
assertEquals(
"unexpected error(s) : " +
Joiner.on(", ").join(compiler.getErrors()),
0, compiler.getErrorCount());
if (descriptions == null) {
assertEquals(
"unexpected warning(s) : " +
Joiner.on(", ").join(compiler.getWarnings()),
0, compiler.getWarningCount());
} else {
assertEquals(
"unexpected warning(s) : " +
Joiner.on(", ").join(compiler.getWarnings()),
descriptions.size(), compiler.getWarningCount());
for (int i = 0; i < descriptions.size(); i++) {
assertEquals(descriptions.get(i),
compiler.getWarnings()[i].description);
}
}
}
void testTypes(String js, String description, boolean isError)
throws Exception {
testTypes(DEFAULT_EXTERNS, js, description, isError);
}
void testTypes(String externs, String js, String description, boolean isError)
throws Exception {
Node n = parseAndTypeCheck(externs, js);
JSError[] errors = compiler.getErrors();
if (description != null && isError) {
assertTrue("expected an error", errors.length > 0);
assertEquals(description, errors[0].description);
errors = Arrays.asList(errors).subList(1, errors.length).toArray(
new JSError[errors.length - 1]);
}
if (errors.length > 0) {
fail("unexpected error(s):\n" + Joiner.on("\n").join(errors));
}
JSError[] warnings = compiler.getWarnings();
if (description != null && !isError) {
assertTrue("expected a warning", warnings.length > 0);
assertEquals(description, warnings[0].description);
warnings = Arrays.asList(warnings).subList(1, warnings.length).toArray(
new JSError[warnings.length - 1]);
}
if (warnings.length > 0) {
fail("unexpected warnings(s):\n" + Joiner.on("\n").join(warnings));
}
}
/**
* Parses and type checks the JavaScript code.
*/
private Node parseAndTypeCheck(String js) {
return parseAndTypeCheck(DEFAULT_EXTERNS, js);
}
private Node parseAndTypeCheck(String externs, String js) {
return parseAndTypeCheckWithScope(externs, js).root;
}
/**
* Parses and type checks the JavaScript code and returns the Scope used
* whilst type checking.
*/
private TypeCheckResult parseAndTypeCheckWithScope(String js) {
return parseAndTypeCheckWithScope(DEFAULT_EXTERNS, js);
}
private TypeCheckResult parseAndTypeCheckWithScope(
String externs, String js) {
compiler.init(
Lists.newArrayList(SourceFile.fromCode("[externs]", externs)),
Lists.newArrayList(SourceFile.fromCode("[testcode]", js)),
compiler.getOptions());
Node n = compiler.getInput(new InputId("[testcode]")).getAstRoot(compiler);
Node externsNode = compiler.getInput(new InputId("[externs]"))
.getAstRoot(compiler);
Node externAndJsRoot = new Node(Token.BLOCK, externsNode, n);
externAndJsRoot.setIsSyntheticBlock(true);
assertEquals("parsing error: " +
Joiner.on(", ").join(compiler.getErrors()),
0, compiler.getErrorCount());
Scope s = makeTypeCheck().processForTesting(externsNode, n);
return new TypeCheckResult(n, s);
}
private Node typeCheck(Node n) {
Node externsNode = new Node(Token.BLOCK);
Node externAndJsRoot = new Node(Token.BLOCK, externsNode, n);
externAndJsRoot.setIsSyntheticBlock(true);
makeTypeCheck().processForTesting(null, n);
return n;
}
private TypeCheck makeTypeCheck() {
return new TypeCheck(
compiler,
new SemanticReverseAbstractInterpreter(
compiler.getCodingConvention(), registry),
registry,
reportMissingOverrides,
CheckLevel.OFF);
}
void testTypes(String js, String[] warnings) throws Exception {
Node n = compiler.parseTestCode(js);
assertEquals(0, compiler.getErrorCount());
Node externsNode = new Node(Token.BLOCK);
Node externAndJsRoot = new Node(Token.BLOCK, externsNode, n);
makeTypeCheck().processForTesting(null, n);
assertEquals(0, compiler.getErrorCount());
if (warnings != null) {
assertEquals(warnings.length, compiler.getWarningCount());
JSError[] messages = compiler.getWarnings();
for (int i = 0; i < warnings.length && i < compiler.getWarningCount();
i++) {
assertEquals(warnings[i], messages[i].description);
}
} else {
assertEquals(0, compiler.getWarningCount());
}
}
String suppressMissingProperty(String ... props) {
String result = "function dummy(x) { ";
for (String prop : props) {
result += "x." + prop + " = 3;";
}
return result + "}";
}
private static class TypeCheckResult {
private final Node root;
private final Scope scope;
private TypeCheckResult(Node root, Scope scope) {
this.root = root;
this.scope = scope;
}
}
} |
||
public void testNestedTypeCheck1735() throws Exception
{
try {
MAPPER.readValue(aposToQuotes(
"{'w':{'type':'java.util.HashMap<java.lang.String,java.lang.String>'}}"),
Wrapper1735.class);
fail("Should not pass");
} catch (JsonMappingException e) {
verifyException(e, "not subtype of");
}
} | com.fasterxml.jackson.databind.jsontype.GenericTypeId1735Test::testNestedTypeCheck1735 | src/test/java/com/fasterxml/jackson/databind/jsontype/GenericTypeId1735Test.java | 62 | src/test/java/com/fasterxml/jackson/databind/jsontype/GenericTypeId1735Test.java | testNestedTypeCheck1735 | package com.fasterxml.jackson.databind.jsontype;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
import com.fasterxml.jackson.databind.*;
// for [databind#1735]:
public class GenericTypeId1735Test extends BaseMapTest
{
static class Wrapper1735 {
@JsonTypeInfo(use = JsonTypeInfo.Id.CLASS, property = "type")
public Payload1735 w;
}
static class Payload1735 {
public void setValue(String str) { }
}
static class Nefarious1735 {
public Nefarious1735() {
throw new Error("Never call this constructor");
}
public void setValue(String str) {
throw new Error("Never call this setter");
}
}
/*
/**********************************************************
/* Unit tests
/**********************************************************
*/
private final ObjectMapper MAPPER = objectMapper();
private final static String NEF_CLASS = Nefarious1735.class.getName();
// Existing checks should kick in fine
public void testSimpleTypeCheck1735() throws Exception
{
try {
MAPPER.readValue(aposToQuotes(
"{'w':{'type':'"+NEF_CLASS+"'}}"),
Wrapper1735.class);
fail("Should not pass");
} catch (JsonMappingException e) {
verifyException(e, "not subtype of");
}
}
// but this was not being verified early enough
public void testNestedTypeCheck1735() throws Exception
{
try {
MAPPER.readValue(aposToQuotes(
"{'w':{'type':'java.util.HashMap<java.lang.String,java.lang.String>'}}"),
Wrapper1735.class);
fail("Should not pass");
} catch (JsonMappingException e) {
verifyException(e, "not subtype of");
}
}
} | // You are a professional Java test case writer, please create a test case named `testNestedTypeCheck1735` for the issue `JacksonDatabind-1735`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonDatabind-1735
//
// ## Issue-Title:
// Missing type checks when using polymorphic type ids
//
// ## Issue-Description:
// (report by Lukes Euler)
//
//
// `JavaType` supports limited amount of generic typing for textual representation, originally just to support typing needed for `EnumMap` (I think). Based on some reports, it appears that some of type compatibility checks are not performed in those cases; if so, they should be made since there is potential for abuse.
//
// The problem here although actual type assignment will fail later on, ability to trigger some of processing (instantiation of incompatible classes, perhaps assingnment of properties) may itself be vulnerability.
//
//
//
//
public void testNestedTypeCheck1735() throws Exception {
| 62 | // but this was not being verified early enough | 88 | 52 | src/test/java/com/fasterxml/jackson/databind/jsontype/GenericTypeId1735Test.java | src/test/java | ```markdown
## Issue-ID: JacksonDatabind-1735
## Issue-Title:
Missing type checks when using polymorphic type ids
## Issue-Description:
(report by Lukes Euler)
`JavaType` supports limited amount of generic typing for textual representation, originally just to support typing needed for `EnumMap` (I think). Based on some reports, it appears that some of type compatibility checks are not performed in those cases; if so, they should be made since there is potential for abuse.
The problem here although actual type assignment will fail later on, ability to trigger some of processing (instantiation of incompatible classes, perhaps assingnment of properties) may itself be vulnerability.
```
You are a professional Java test case writer, please create a test case named `testNestedTypeCheck1735` for the issue `JacksonDatabind-1735`, utilizing the provided issue report information and the following function signature.
```java
public void testNestedTypeCheck1735() throws Exception {
```
| 52 | [
"com.fasterxml.jackson.databind.jsontype.impl.ClassNameIdResolver"
] | 798076d44fe7545aceaa07a180af8314e184b1e6ae2f687a4c4df4b2fc75def2 | public void testNestedTypeCheck1735() throws Exception
| // You are a professional Java test case writer, please create a test case named `testNestedTypeCheck1735` for the issue `JacksonDatabind-1735`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonDatabind-1735
//
// ## Issue-Title:
// Missing type checks when using polymorphic type ids
//
// ## Issue-Description:
// (report by Lukes Euler)
//
//
// `JavaType` supports limited amount of generic typing for textual representation, originally just to support typing needed for `EnumMap` (I think). Based on some reports, it appears that some of type compatibility checks are not performed in those cases; if so, they should be made since there is potential for abuse.
//
// The problem here although actual type assignment will fail later on, ability to trigger some of processing (instantiation of incompatible classes, perhaps assingnment of properties) may itself be vulnerability.
//
//
//
//
| JacksonDatabind | package com.fasterxml.jackson.databind.jsontype;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
import com.fasterxml.jackson.databind.*;
// for [databind#1735]:
public class GenericTypeId1735Test extends BaseMapTest
{
static class Wrapper1735 {
@JsonTypeInfo(use = JsonTypeInfo.Id.CLASS, property = "type")
public Payload1735 w;
}
static class Payload1735 {
public void setValue(String str) { }
}
static class Nefarious1735 {
public Nefarious1735() {
throw new Error("Never call this constructor");
}
public void setValue(String str) {
throw new Error("Never call this setter");
}
}
/*
/**********************************************************
/* Unit tests
/**********************************************************
*/
private final ObjectMapper MAPPER = objectMapper();
private final static String NEF_CLASS = Nefarious1735.class.getName();
// Existing checks should kick in fine
public void testSimpleTypeCheck1735() throws Exception
{
try {
MAPPER.readValue(aposToQuotes(
"{'w':{'type':'"+NEF_CLASS+"'}}"),
Wrapper1735.class);
fail("Should not pass");
} catch (JsonMappingException e) {
verifyException(e, "not subtype of");
}
}
// but this was not being verified early enough
public void testNestedTypeCheck1735() throws Exception
{
try {
MAPPER.readValue(aposToQuotes(
"{'w':{'type':'java.util.HashMap<java.lang.String,java.lang.String>'}}"),
Wrapper1735.class);
fail("Should not pass");
} catch (JsonMappingException e) {
verifyException(e, "not subtype of");
}
}
} |
|
public void testExpressionInForIn() {
assertLiveBeforeX("var a = [0]; X:for (a[1] in foo) { }", "a");
} | com.google.javascript.jscomp.LiveVariableAnalysisTest::testExpressionInForIn | test/com/google/javascript/jscomp/LiveVariableAnalysisTest.java | 210 | test/com/google/javascript/jscomp/LiveVariableAnalysisTest.java | testExpressionInForIn | /*
* Copyright 2008 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import com.google.javascript.jscomp.DataFlowAnalysis.FlowState;
import com.google.javascript.jscomp.Scope.Var;
import com.google.javascript.rhino.InputId;
import com.google.javascript.rhino.Node;
import com.google.javascript.rhino.Token;
import junit.framework.TestCase;
/**
* Tests for {@link LiveVariablesAnalysis}. Test cases are snippets of a
* function and assertions are made at the instruction labeled with {@code X}.
*
*/
public class LiveVariableAnalysisTest extends TestCase {
private LiveVariablesAnalysis liveness = null;
public void testStraightLine() {
// A sample of simple straight line of code with different liveness changes.
assertNotLiveBeforeX("X:var a;", "a");
assertNotLiveAfterX("X:var a;", "a");
assertNotLiveAfterX("X:var a=1;", "a");
assertLiveAfterX("X:var a=1; a()", "a");
assertNotLiveBeforeX("X:var a=1; a()", "a");
assertLiveBeforeX("var a;X:a;", "a");
assertLiveBeforeX("var a;X:a=a+1;", "a");
assertLiveBeforeX("var a;X:a+=1;", "a");
assertLiveBeforeX("var a;X:a++;", "a");
assertNotLiveAfterX("var a,b;X:b();", "a");
assertNotLiveBeforeX("var a,b;X:b();", "a");
assertLiveBeforeX("var a,b;X:b(a);", "a");
assertLiveBeforeX("var a,b;X:b(1,2,3,b(a + 1));", "a");
assertNotLiveBeforeX("var a,b;X:a=1;b(a)", "a");
assertNotLiveAfterX("var a,b;X:b(a);b()", "a");
assertLiveBeforeX("var a,b;X:b();b=1;a()", "b");
assertLiveAfterX("X:a();var a;a()", "a");
assertNotLiveAfterX("X:a();var a=1;a()", "a");
assertLiveBeforeX("var a,b;X:a,b=1", "a");
}
public void testProperties() {
// Reading property of a local variable makes that variable live.
assertLiveBeforeX("var a,b;X:a.P;", "a");
// Assigning to a property doesn't kill "a". It makes it live instead.
assertLiveBeforeX("var a,b;X:a.P=1;b()", "a");
assertLiveBeforeX("var a,b;X:a.P.Q=1;b()", "a");
// An "a" in a different context.
assertNotLiveAfterX("var a,b;X:b.P.Q.a=1;", "a");
assertLiveBeforeX("var a,b;X:b.P.Q=a;", "a");
}
public void testConditions() {
// Reading the condition makes the variable live.
assertLiveBeforeX("var a,b;X:if(a){}", "a");
assertLiveBeforeX("var a,b;X:if(a||b) {}", "a");
assertLiveBeforeX("var a,b;X:if(b||a) {}", "a");
assertLiveBeforeX("var a,b;X:if(b||b(a)) {}", "a");
assertNotLiveAfterX("var a,b;X:b();if(a) {}", "b");
// We can kill within a condition as well.
assertNotLiveAfterX("var a,b;X:a();if(a=b){}a()", "a");
assertNotLiveAfterX("var a,b;X:a();while(a=b){}a()", "a");
// The kill can be "conditional" due to short circuit.
assertNotLiveAfterX("var a,b;X:a();if((a=b)&&b){}a()", "a");
assertNotLiveAfterX("var a,b;X:a();while((a=b)&&b){}a()", "a");
assertLiveBeforeX("var a,b;a();X:if(b&&(a=b)){}a()", "a"); // Assumed live.
assertLiveBeforeX("var a,b;a();X:if(a&&(a=b)){}a()", "a");
assertLiveBeforeX("var a,b;a();X:while(b&&(a=b)){}a()", "a");
assertLiveBeforeX("var a,b;a();X:while(a&&(a=b)){}a()", "a");
}
public void testArrays() {
assertLiveBeforeX("var a;X:a[1]", "a");
assertLiveBeforeX("var a,b;X:b[a]", "a");
assertLiveBeforeX("var a,b;X:b[1,2,3,4,b(a)]", "a");
assertLiveBeforeX("var a,b;X:b=[a,'a']", "a");
assertNotLiveBeforeX("var a,b;X:a=[];b(a)", "a");
// Element assignment doesn't kill the array.
assertLiveBeforeX("var a;X:a[1]=1", "a");
}
public void testTwoPaths() {
// Both Paths.
assertLiveBeforeX("var a,b;X:if(b){b(a)}else{b(a)};", "a");
// Only one path.
assertLiveBeforeX("var a,b;X:if(b){b(b)}else{b(a)};", "a");
assertLiveBeforeX("var a,b;X:if(b){b(a)}else{b(b)};", "a");
// None of the paths.
assertNotLiveAfterX("var a,b;X:if(b){b(b)}else{b(b)};", "a");
// At the very end.
assertLiveBeforeX("var a,b;X:if(b){b(b)}else{b(b)}a();", "a");
// The loop might or might not be executed.
assertLiveBeforeX("var a;X:while(param1){a()};", "a");
assertLiveBeforeX("var a;X:while(param1){a=1};a()", "a");
// Same idea with if.
assertLiveBeforeX("var a;X:if(param1){a()};", "a");
assertLiveBeforeX("var a;X:if(param1){a=1};a()", "a");
// This is different in DO. We know for sure at least one iteration is
// executed.
assertNotLiveAfterX("X:var a;do{a=1}while(param1);a()", "a");
}
public void testThreePaths() {
assertLiveBeforeX("var a;X:if(1){}else if(2){}else{a()};", "a");
assertLiveBeforeX("var a;X:if(1){}else if(2){a()}else{};", "a");
assertLiveBeforeX("var a;X:if(1){a()}else if(2){}else{};", "a");
assertLiveBeforeX("var a;X:if(1){}else if(2){}else{};a()", "a");
}
public void testHooks() {
assertLiveBeforeX("var a;X:1?a=1:1;a()", "a");
// Unfortunately, we cannot prove the following because we assume there is
// no control flow within a hook (ie: no joins / set unions).
// assertNotLiveAfterX("var a;X:1?a=1:a=2;a", "a");
assertLiveBeforeX("var a,b;X:b=1?a:2", "a");
}
public void testForLoops() {
// Induction variable should not be live after the loop.
assertNotLiveBeforeX("var a,b;for(a=0;a<9;a++){b(a)};X:b", "a");
assertNotLiveBeforeX("var a,b;for(a in b){a()};X:b", "a");
assertNotLiveBeforeX("var a,b;for(a in b){a()};X:a", "b");
assertLiveBeforeX("var b;for(var a in b){X:a()};", "a");
// It should be live within the loop even if it is not used.
assertLiveBeforeX("var a,b;for(a=0;a<9;a++){X:1}", "a");
assertLiveAfterX("var a,b;for(a in b){X:b};", "a");
// For-In should serve as a gen as well.
assertLiveBeforeX("var a,b; X:for(a in b){ }", "a");
// "a in b" should kill "a" before it.
// Can't prove this unless we have branched backward DFA.
//assertNotLiveAfterX("var a,b;X:b;for(a in b){a()};", "a");
// Unless it is used before.
assertLiveBeforeX("var a,b;X:a();b();for(a in b){a()};", "a");
// Initializer
assertLiveBeforeX("var a,b;X:b;for(b=a;;){};", "a");
assertNotLiveBeforeX("var a,b;X:a;for(b=a;;){b()};b();", "b");
}
public void testNestedLoops() {
assertLiveBeforeX("var a;X:while(1){while(1){a()}}", "a");
assertLiveBeforeX("var a;X:while(1){while(1){while(1){a()}}}", "a");
assertLiveBeforeX("var a;X:while(1){while(1){a()};a=1}", "a");
assertLiveAfterX("var a;while(1){while(1){a()};X:a=1;}", "a");
assertLiveAfterX("var a;while(1){X:a=1;while(1){a()}}", "a");
assertNotLiveBeforeX(
"var a;X:1;do{do{do{a=1;}while(1)}while(1)}while(1);a()", "a");
}
public void testSwitches() {
assertLiveBeforeX("var a,b;X:switch(a){}", "a");
assertLiveBeforeX("var a,b;X:switch(b){case(a):break;}", "a");
assertLiveBeforeX("var a,b;X:switch(b){case(b):case(a):break;}", "a");
assertNotLiveBeforeX(
"var a,b;X:switch(b){case 1:a=1;break;default:a=2;break};a()", "a");
assertLiveBeforeX("var a,b;X:switch(b){default:a();break;}", "a");
}
public void testAssignAndReadInCondition() {
// BUG #1358904
// Technically, this isn't exactly true....but we haven't model control flow
// within an instruction.
assertLiveBeforeX("var a, b; X: if ((a = this) && (b = a)) {}", "a");
assertNotLiveBeforeX("var a, b; X: a = 1, b = 1;", "a");
assertNotLiveBeforeX("var a; X: a = 1, a = 1;", "a");
}
public void testParam() {
// Unused parameter should not be live.
assertNotLiveAfterX("var a;X:a()", "param1");
assertLiveBeforeX("var a;X:a(param1)", "param1");
assertNotLiveAfterX("var a;X:a();a(param2)", "param1");
}
public void testExpressionInForIn() {
assertLiveBeforeX("var a = [0]; X:for (a[1] in foo) { }", "a");
}
public void testArgumentsArray() {
// Check that use of arguments forces the parameters into the
// escaped set.
assertEscaped("arguments[0]", "param1");
assertEscaped("arguments[0]", "param2");
assertEscaped("var args = arguments", "param1");
assertEscaped("var args = arguments", "param2");
assertNotEscaped("arguments = []", "param1");
assertNotEscaped("arguments = []", "param2");
assertEscaped("arguments[0] = 1", "param1");
assertEscaped("arguments[0] = 1", "param2");
assertEscaped("arguments[arguments[0]] = 1", "param1");
assertEscaped("arguments[arguments[0]] = 1", "param2");
}
public void testTryCatchFinally() {
assertLiveAfterX("var a; try {X:a=1} finally {a}", "a");
assertLiveAfterX("var a; try {a()} catch(e) {X:a=1} finally {a}", "a");
// Because the outer catch doesn't catch any exceptions at all, the read of
// "a" within the catch block should not make "a" live.
assertNotLiveAfterX("var a = 1; try {" +
"try {a()} catch(e) {X:1} } catch(E) {a}", "a");
assertLiveAfterX("var a; while(1) { try {X:a=1;break} finally {a}}", "a");
}
public void testExceptionThrowingAssignments() {
assertLiveBeforeX("try{var a; X:a=foo();a} catch(e) {e()}", "a");
assertLiveBeforeX("try{X:var a=foo();a} catch(e) {e()}", "a");
assertLiveBeforeX("try{X:var a=foo()} catch(e) {e(a)}", "a");
}
public void testInnerFunctions() {
assertLiveBeforeX("function a() {}; X: a()", "a");
assertNotLiveBeforeX("X: function a() {}", "a");
assertLiveBeforeX("a = function(){}; function a() {}; X: a()", "a");
// NOTE: function a() {} has no CFG node representation since it is not
// part of the control execution.
assertLiveAfterX("X: a = function(){}; function a() {}; a()", "a");
assertNotLiveBeforeX("X: a = function(){}; function a() {}; a()", "a");
}
public void testEscaped() {
assertEscaped("var a;function b(){a()}", "a");
assertEscaped("var a;function b(){param1()}", "param1");
assertEscaped("var a;function b(){function c(){a()}}", "a");
assertEscaped("var a;function b(){param1.x = function() {a()}}", "a");
assertEscaped("try{} catch(e){}", "e");
assertNotEscaped("var a;function b(){var c; c()}", "c");
assertNotEscaped("var a;function f(){function b(){var c;c()}}", "c");
assertNotEscaped("var a;function b(){};a()", "a");
assertNotEscaped("var a;function f(){function b(){}}a()", "a");
assertNotEscaped("var a;function b(){var a;a()};a()", "a");
// Escaped by exporting.
assertEscaped("var _x", "_x");
}
public void testEscapedLiveness() {
assertNotLiveBeforeX("var a;X:a();function b(){a()}", "a");
}
public void testBug1449316() {
assertLiveBeforeX("try {var x=[]; X:var y=x[0]} finally {foo()}", "x");
}
private void assertLiveBeforeX(String src, String var) {
FlowState<LiveVariablesAnalysis.LiveVariableLattice> state =
getFlowStateAtX(src);
assertNotNull(src + " should contain a label 'X:'", state);
assertTrue("Variable" + var + " should be live before X", state.getIn()
.isLive(liveness.getVarIndex(var)));
}
private void assertLiveAfterX(String src, String var) {
FlowState<LiveVariablesAnalysis.LiveVariableLattice> state =
getFlowStateAtX(src);
assertTrue("Label X should be in the input program.", state != null);
assertTrue("Variable" + var + " should be live after X", state.getOut()
.isLive(liveness.getVarIndex(var)));
}
private void assertNotLiveAfterX(String src, String var) {
FlowState<LiveVariablesAnalysis.LiveVariableLattice> state =
getFlowStateAtX(src);
assertTrue("Label X should be in the input program.", state != null);
assertTrue("Variable" + var + " should not be live after X", !state
.getOut().isLive(liveness.getVarIndex(var)));
}
private void assertNotLiveBeforeX(String src, String var) {
FlowState<LiveVariablesAnalysis.LiveVariableLattice> state =
getFlowStateAtX(src);
assertTrue("Label X should be in the input program.", state != null);
assertTrue("Variable" + var + " should not be live before X", !state
.getIn().isLive(liveness.getVarIndex(var)));
}
private FlowState<LiveVariablesAnalysis.LiveVariableLattice> getFlowStateAtX(
String src) {
liveness = computeLiveness(src);
return getFlowStateAtX(liveness.getCfg().getEntry().getValue(), liveness
.getCfg());
}
private FlowState<LiveVariablesAnalysis.LiveVariableLattice> getFlowStateAtX(
Node node, ControlFlowGraph<Node> cfg) {
if (node.getType() == Token.LABEL) {
if (node.getFirstChild().getString().equals("X")) {
return cfg.getNode(node.getLastChild()).getAnnotation();
}
}
for (Node c = node.getFirstChild(); c != null; c = c.getNext()) {
FlowState<LiveVariablesAnalysis.LiveVariableLattice> state =
getFlowStateAtX(c, cfg);
if (state != null) {
return state;
}
}
return null;
}
private static void assertEscaped(String src, String name) {
for (Var var : computeLiveness(src).getEscapedLocals()) {
if (var.name.equals(name)) {
return;
}
}
fail("Variable " + name + " should be in the escaped local list.");
}
private static void assertNotEscaped(String src, String name) {
for (Var var : computeLiveness(src).getEscapedLocals()) {
assertFalse(var.name.equals(name));
}
}
private static LiveVariablesAnalysis computeLiveness(String src) {
Compiler compiler = new Compiler();
CompilerOptions options = new CompilerOptions();
options.setCodingConvention(new GoogleCodingConvention());
compiler.initOptions(options);
src = "function _FUNCTION(param1, param2){" + src + "}";
Node n = compiler.parseTestCode(src).removeFirstChild();
Node script = new Node(Token.SCRIPT, n);
script.setInputId(new InputId("test"));
assertEquals(0, compiler.getErrorCount());
Scope scope = new SyntacticScopeCreator(compiler).createScope(
n, new Scope(script, compiler));
ControlFlowAnalysis cfa = new ControlFlowAnalysis(compiler, false, true);
cfa.process(null, n);
ControlFlowGraph<Node> cfg = cfa.getCfg();
LiveVariablesAnalysis analysis =
new LiveVariablesAnalysis(cfg, scope, compiler);
analysis.analyze();
return analysis;
}
} | // You are a professional Java test case writer, please create a test case named `testExpressionInForIn` for the issue `Closure-528`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-528
//
// ## Issue-Title:
// Online CC bug: report java error.
//
// ## Issue-Description:
// **What steps will reproduce the problem?**
// 1. open http://closure-compiler.appspot.com/
// 2. input js code:
// function keys(obj) {
// var a = [], i = 0;
// for (a[i++] in obj)
// ;
// return a;
// }
// 3. press [compile] button.
//
// **What is the expected output? What do you see instead?**
// Except OK. See java error.
//
// **What version of the product are you using? On what operating system?**
// Online CC version.
//
//
public void testExpressionInForIn() {
| 210 | 58 | 208 | test/com/google/javascript/jscomp/LiveVariableAnalysisTest.java | test | ```markdown
## Issue-ID: Closure-528
## Issue-Title:
Online CC bug: report java error.
## Issue-Description:
**What steps will reproduce the problem?**
1. open http://closure-compiler.appspot.com/
2. input js code:
function keys(obj) {
var a = [], i = 0;
for (a[i++] in obj)
;
return a;
}
3. press [compile] button.
**What is the expected output? What do you see instead?**
Except OK. See java error.
**What version of the product are you using? On what operating system?**
Online CC version.
```
You are a professional Java test case writer, please create a test case named `testExpressionInForIn` for the issue `Closure-528`, utilizing the provided issue report information and the following function signature.
```java
public void testExpressionInForIn() {
```
| 208 | [
"com.google.javascript.jscomp.LiveVariablesAnalysis"
] | 799dee72411592e340952ee55b93d630633b9c48d0927e2660b55134298b83fa | public void testExpressionInForIn() | // You are a professional Java test case writer, please create a test case named `testExpressionInForIn` for the issue `Closure-528`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-528
//
// ## Issue-Title:
// Online CC bug: report java error.
//
// ## Issue-Description:
// **What steps will reproduce the problem?**
// 1. open http://closure-compiler.appspot.com/
// 2. input js code:
// function keys(obj) {
// var a = [], i = 0;
// for (a[i++] in obj)
// ;
// return a;
// }
// 3. press [compile] button.
//
// **What is the expected output? What do you see instead?**
// Except OK. See java error.
//
// **What version of the product are you using? On what operating system?**
// Online CC version.
//
//
| Closure | /*
* Copyright 2008 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import com.google.javascript.jscomp.DataFlowAnalysis.FlowState;
import com.google.javascript.jscomp.Scope.Var;
import com.google.javascript.rhino.InputId;
import com.google.javascript.rhino.Node;
import com.google.javascript.rhino.Token;
import junit.framework.TestCase;
/**
* Tests for {@link LiveVariablesAnalysis}. Test cases are snippets of a
* function and assertions are made at the instruction labeled with {@code X}.
*
*/
public class LiveVariableAnalysisTest extends TestCase {
private LiveVariablesAnalysis liveness = null;
public void testStraightLine() {
// A sample of simple straight line of code with different liveness changes.
assertNotLiveBeforeX("X:var a;", "a");
assertNotLiveAfterX("X:var a;", "a");
assertNotLiveAfterX("X:var a=1;", "a");
assertLiveAfterX("X:var a=1; a()", "a");
assertNotLiveBeforeX("X:var a=1; a()", "a");
assertLiveBeforeX("var a;X:a;", "a");
assertLiveBeforeX("var a;X:a=a+1;", "a");
assertLiveBeforeX("var a;X:a+=1;", "a");
assertLiveBeforeX("var a;X:a++;", "a");
assertNotLiveAfterX("var a,b;X:b();", "a");
assertNotLiveBeforeX("var a,b;X:b();", "a");
assertLiveBeforeX("var a,b;X:b(a);", "a");
assertLiveBeforeX("var a,b;X:b(1,2,3,b(a + 1));", "a");
assertNotLiveBeforeX("var a,b;X:a=1;b(a)", "a");
assertNotLiveAfterX("var a,b;X:b(a);b()", "a");
assertLiveBeforeX("var a,b;X:b();b=1;a()", "b");
assertLiveAfterX("X:a();var a;a()", "a");
assertNotLiveAfterX("X:a();var a=1;a()", "a");
assertLiveBeforeX("var a,b;X:a,b=1", "a");
}
public void testProperties() {
// Reading property of a local variable makes that variable live.
assertLiveBeforeX("var a,b;X:a.P;", "a");
// Assigning to a property doesn't kill "a". It makes it live instead.
assertLiveBeforeX("var a,b;X:a.P=1;b()", "a");
assertLiveBeforeX("var a,b;X:a.P.Q=1;b()", "a");
// An "a" in a different context.
assertNotLiveAfterX("var a,b;X:b.P.Q.a=1;", "a");
assertLiveBeforeX("var a,b;X:b.P.Q=a;", "a");
}
public void testConditions() {
// Reading the condition makes the variable live.
assertLiveBeforeX("var a,b;X:if(a){}", "a");
assertLiveBeforeX("var a,b;X:if(a||b) {}", "a");
assertLiveBeforeX("var a,b;X:if(b||a) {}", "a");
assertLiveBeforeX("var a,b;X:if(b||b(a)) {}", "a");
assertNotLiveAfterX("var a,b;X:b();if(a) {}", "b");
// We can kill within a condition as well.
assertNotLiveAfterX("var a,b;X:a();if(a=b){}a()", "a");
assertNotLiveAfterX("var a,b;X:a();while(a=b){}a()", "a");
// The kill can be "conditional" due to short circuit.
assertNotLiveAfterX("var a,b;X:a();if((a=b)&&b){}a()", "a");
assertNotLiveAfterX("var a,b;X:a();while((a=b)&&b){}a()", "a");
assertLiveBeforeX("var a,b;a();X:if(b&&(a=b)){}a()", "a"); // Assumed live.
assertLiveBeforeX("var a,b;a();X:if(a&&(a=b)){}a()", "a");
assertLiveBeforeX("var a,b;a();X:while(b&&(a=b)){}a()", "a");
assertLiveBeforeX("var a,b;a();X:while(a&&(a=b)){}a()", "a");
}
public void testArrays() {
assertLiveBeforeX("var a;X:a[1]", "a");
assertLiveBeforeX("var a,b;X:b[a]", "a");
assertLiveBeforeX("var a,b;X:b[1,2,3,4,b(a)]", "a");
assertLiveBeforeX("var a,b;X:b=[a,'a']", "a");
assertNotLiveBeforeX("var a,b;X:a=[];b(a)", "a");
// Element assignment doesn't kill the array.
assertLiveBeforeX("var a;X:a[1]=1", "a");
}
public void testTwoPaths() {
// Both Paths.
assertLiveBeforeX("var a,b;X:if(b){b(a)}else{b(a)};", "a");
// Only one path.
assertLiveBeforeX("var a,b;X:if(b){b(b)}else{b(a)};", "a");
assertLiveBeforeX("var a,b;X:if(b){b(a)}else{b(b)};", "a");
// None of the paths.
assertNotLiveAfterX("var a,b;X:if(b){b(b)}else{b(b)};", "a");
// At the very end.
assertLiveBeforeX("var a,b;X:if(b){b(b)}else{b(b)}a();", "a");
// The loop might or might not be executed.
assertLiveBeforeX("var a;X:while(param1){a()};", "a");
assertLiveBeforeX("var a;X:while(param1){a=1};a()", "a");
// Same idea with if.
assertLiveBeforeX("var a;X:if(param1){a()};", "a");
assertLiveBeforeX("var a;X:if(param1){a=1};a()", "a");
// This is different in DO. We know for sure at least one iteration is
// executed.
assertNotLiveAfterX("X:var a;do{a=1}while(param1);a()", "a");
}
public void testThreePaths() {
assertLiveBeforeX("var a;X:if(1){}else if(2){}else{a()};", "a");
assertLiveBeforeX("var a;X:if(1){}else if(2){a()}else{};", "a");
assertLiveBeforeX("var a;X:if(1){a()}else if(2){}else{};", "a");
assertLiveBeforeX("var a;X:if(1){}else if(2){}else{};a()", "a");
}
public void testHooks() {
assertLiveBeforeX("var a;X:1?a=1:1;a()", "a");
// Unfortunately, we cannot prove the following because we assume there is
// no control flow within a hook (ie: no joins / set unions).
// assertNotLiveAfterX("var a;X:1?a=1:a=2;a", "a");
assertLiveBeforeX("var a,b;X:b=1?a:2", "a");
}
public void testForLoops() {
// Induction variable should not be live after the loop.
assertNotLiveBeforeX("var a,b;for(a=0;a<9;a++){b(a)};X:b", "a");
assertNotLiveBeforeX("var a,b;for(a in b){a()};X:b", "a");
assertNotLiveBeforeX("var a,b;for(a in b){a()};X:a", "b");
assertLiveBeforeX("var b;for(var a in b){X:a()};", "a");
// It should be live within the loop even if it is not used.
assertLiveBeforeX("var a,b;for(a=0;a<9;a++){X:1}", "a");
assertLiveAfterX("var a,b;for(a in b){X:b};", "a");
// For-In should serve as a gen as well.
assertLiveBeforeX("var a,b; X:for(a in b){ }", "a");
// "a in b" should kill "a" before it.
// Can't prove this unless we have branched backward DFA.
//assertNotLiveAfterX("var a,b;X:b;for(a in b){a()};", "a");
// Unless it is used before.
assertLiveBeforeX("var a,b;X:a();b();for(a in b){a()};", "a");
// Initializer
assertLiveBeforeX("var a,b;X:b;for(b=a;;){};", "a");
assertNotLiveBeforeX("var a,b;X:a;for(b=a;;){b()};b();", "b");
}
public void testNestedLoops() {
assertLiveBeforeX("var a;X:while(1){while(1){a()}}", "a");
assertLiveBeforeX("var a;X:while(1){while(1){while(1){a()}}}", "a");
assertLiveBeforeX("var a;X:while(1){while(1){a()};a=1}", "a");
assertLiveAfterX("var a;while(1){while(1){a()};X:a=1;}", "a");
assertLiveAfterX("var a;while(1){X:a=1;while(1){a()}}", "a");
assertNotLiveBeforeX(
"var a;X:1;do{do{do{a=1;}while(1)}while(1)}while(1);a()", "a");
}
public void testSwitches() {
assertLiveBeforeX("var a,b;X:switch(a){}", "a");
assertLiveBeforeX("var a,b;X:switch(b){case(a):break;}", "a");
assertLiveBeforeX("var a,b;X:switch(b){case(b):case(a):break;}", "a");
assertNotLiveBeforeX(
"var a,b;X:switch(b){case 1:a=1;break;default:a=2;break};a()", "a");
assertLiveBeforeX("var a,b;X:switch(b){default:a();break;}", "a");
}
public void testAssignAndReadInCondition() {
// BUG #1358904
// Technically, this isn't exactly true....but we haven't model control flow
// within an instruction.
assertLiveBeforeX("var a, b; X: if ((a = this) && (b = a)) {}", "a");
assertNotLiveBeforeX("var a, b; X: a = 1, b = 1;", "a");
assertNotLiveBeforeX("var a; X: a = 1, a = 1;", "a");
}
public void testParam() {
// Unused parameter should not be live.
assertNotLiveAfterX("var a;X:a()", "param1");
assertLiveBeforeX("var a;X:a(param1)", "param1");
assertNotLiveAfterX("var a;X:a();a(param2)", "param1");
}
public void testExpressionInForIn() {
assertLiveBeforeX("var a = [0]; X:for (a[1] in foo) { }", "a");
}
public void testArgumentsArray() {
// Check that use of arguments forces the parameters into the
// escaped set.
assertEscaped("arguments[0]", "param1");
assertEscaped("arguments[0]", "param2");
assertEscaped("var args = arguments", "param1");
assertEscaped("var args = arguments", "param2");
assertNotEscaped("arguments = []", "param1");
assertNotEscaped("arguments = []", "param2");
assertEscaped("arguments[0] = 1", "param1");
assertEscaped("arguments[0] = 1", "param2");
assertEscaped("arguments[arguments[0]] = 1", "param1");
assertEscaped("arguments[arguments[0]] = 1", "param2");
}
public void testTryCatchFinally() {
assertLiveAfterX("var a; try {X:a=1} finally {a}", "a");
assertLiveAfterX("var a; try {a()} catch(e) {X:a=1} finally {a}", "a");
// Because the outer catch doesn't catch any exceptions at all, the read of
// "a" within the catch block should not make "a" live.
assertNotLiveAfterX("var a = 1; try {" +
"try {a()} catch(e) {X:1} } catch(E) {a}", "a");
assertLiveAfterX("var a; while(1) { try {X:a=1;break} finally {a}}", "a");
}
public void testExceptionThrowingAssignments() {
assertLiveBeforeX("try{var a; X:a=foo();a} catch(e) {e()}", "a");
assertLiveBeforeX("try{X:var a=foo();a} catch(e) {e()}", "a");
assertLiveBeforeX("try{X:var a=foo()} catch(e) {e(a)}", "a");
}
public void testInnerFunctions() {
assertLiveBeforeX("function a() {}; X: a()", "a");
assertNotLiveBeforeX("X: function a() {}", "a");
assertLiveBeforeX("a = function(){}; function a() {}; X: a()", "a");
// NOTE: function a() {} has no CFG node representation since it is not
// part of the control execution.
assertLiveAfterX("X: a = function(){}; function a() {}; a()", "a");
assertNotLiveBeforeX("X: a = function(){}; function a() {}; a()", "a");
}
public void testEscaped() {
assertEscaped("var a;function b(){a()}", "a");
assertEscaped("var a;function b(){param1()}", "param1");
assertEscaped("var a;function b(){function c(){a()}}", "a");
assertEscaped("var a;function b(){param1.x = function() {a()}}", "a");
assertEscaped("try{} catch(e){}", "e");
assertNotEscaped("var a;function b(){var c; c()}", "c");
assertNotEscaped("var a;function f(){function b(){var c;c()}}", "c");
assertNotEscaped("var a;function b(){};a()", "a");
assertNotEscaped("var a;function f(){function b(){}}a()", "a");
assertNotEscaped("var a;function b(){var a;a()};a()", "a");
// Escaped by exporting.
assertEscaped("var _x", "_x");
}
public void testEscapedLiveness() {
assertNotLiveBeforeX("var a;X:a();function b(){a()}", "a");
}
public void testBug1449316() {
assertLiveBeforeX("try {var x=[]; X:var y=x[0]} finally {foo()}", "x");
}
private void assertLiveBeforeX(String src, String var) {
FlowState<LiveVariablesAnalysis.LiveVariableLattice> state =
getFlowStateAtX(src);
assertNotNull(src + " should contain a label 'X:'", state);
assertTrue("Variable" + var + " should be live before X", state.getIn()
.isLive(liveness.getVarIndex(var)));
}
private void assertLiveAfterX(String src, String var) {
FlowState<LiveVariablesAnalysis.LiveVariableLattice> state =
getFlowStateAtX(src);
assertTrue("Label X should be in the input program.", state != null);
assertTrue("Variable" + var + " should be live after X", state.getOut()
.isLive(liveness.getVarIndex(var)));
}
private void assertNotLiveAfterX(String src, String var) {
FlowState<LiveVariablesAnalysis.LiveVariableLattice> state =
getFlowStateAtX(src);
assertTrue("Label X should be in the input program.", state != null);
assertTrue("Variable" + var + " should not be live after X", !state
.getOut().isLive(liveness.getVarIndex(var)));
}
private void assertNotLiveBeforeX(String src, String var) {
FlowState<LiveVariablesAnalysis.LiveVariableLattice> state =
getFlowStateAtX(src);
assertTrue("Label X should be in the input program.", state != null);
assertTrue("Variable" + var + " should not be live before X", !state
.getIn().isLive(liveness.getVarIndex(var)));
}
private FlowState<LiveVariablesAnalysis.LiveVariableLattice> getFlowStateAtX(
String src) {
liveness = computeLiveness(src);
return getFlowStateAtX(liveness.getCfg().getEntry().getValue(), liveness
.getCfg());
}
private FlowState<LiveVariablesAnalysis.LiveVariableLattice> getFlowStateAtX(
Node node, ControlFlowGraph<Node> cfg) {
if (node.getType() == Token.LABEL) {
if (node.getFirstChild().getString().equals("X")) {
return cfg.getNode(node.getLastChild()).getAnnotation();
}
}
for (Node c = node.getFirstChild(); c != null; c = c.getNext()) {
FlowState<LiveVariablesAnalysis.LiveVariableLattice> state =
getFlowStateAtX(c, cfg);
if (state != null) {
return state;
}
}
return null;
}
private static void assertEscaped(String src, String name) {
for (Var var : computeLiveness(src).getEscapedLocals()) {
if (var.name.equals(name)) {
return;
}
}
fail("Variable " + name + " should be in the escaped local list.");
}
private static void assertNotEscaped(String src, String name) {
for (Var var : computeLiveness(src).getEscapedLocals()) {
assertFalse(var.name.equals(name));
}
}
private static LiveVariablesAnalysis computeLiveness(String src) {
Compiler compiler = new Compiler();
CompilerOptions options = new CompilerOptions();
options.setCodingConvention(new GoogleCodingConvention());
compiler.initOptions(options);
src = "function _FUNCTION(param1, param2){" + src + "}";
Node n = compiler.parseTestCode(src).removeFirstChild();
Node script = new Node(Token.SCRIPT, n);
script.setInputId(new InputId("test"));
assertEquals(0, compiler.getErrorCount());
Scope scope = new SyntacticScopeCreator(compiler).createScope(
n, new Scope(script, compiler));
ControlFlowAnalysis cfa = new ControlFlowAnalysis(compiler, false, true);
cfa.process(null, n);
ControlFlowGraph<Node> cfg = cfa.getCfg();
LiveVariablesAnalysis analysis =
new LiveVariablesAnalysis(cfg, scope, compiler);
analysis.analyze();
return analysis;
}
} |
||
public void testMathpbx02() {
double[] mainTridiagonal = {
7484.860960227216, 18405.28129035345, 13855.225609560746,
10016.708722343366, 559.8117399576674, 6750.190788301587,
71.21428769782159
};
double[] secondaryTridiagonal = {
-4175.088570476366,1975.7955858241994,5193.178422374075,
1995.286659169179,75.34535882933804,-234.0808002076056
};
// the reference values have been computed using routine DSTEMR
// from the fortran library LAPACK version 3.2.1
double[] refEigenValues = {
20654.744890306974412,16828.208208485466457,
6893.155912634994820,6757.083016675340332,
5887.799885688558788,64.309089923240379,
57.992628792736340
};
RealVector[] refEigenVectors = {
new ArrayRealVector(new double[] {-0.270356342026904, 0.852811091326997, 0.399639490702077, 0.198794657813990, 0.019739323307666, 0.000106983022327, -0.000001216636321}),
new ArrayRealVector(new double[] {0.179995273578326,-0.402807848153042,0.701870993525734,0.555058211014888,0.068079148898236,0.000509139115227,-0.000007112235617}),
new ArrayRealVector(new double[] {-0.399582721284727,-0.056629954519333,-0.514406488522827,0.711168164518580,0.225548081276367,0.125943999652923,-0.004321507456014}),
new ArrayRealVector(new double[] {0.058515721572821,0.010200130057739,0.063516274916536,-0.090696087449378,-0.017148420432597,0.991318870265707,-0.034707338554096}),
new ArrayRealVector(new double[] {0.855205995537564,0.327134656629775,-0.265382397060548,0.282690729026706,0.105736068025572,-0.009138126622039,0.000367751821196}),
new ArrayRealVector(new double[] {-0.002913069901144,-0.005177515777101,0.041906334478672,-0.109315918416258,0.436192305456741,0.026307315639535,0.891797507436344}),
new ArrayRealVector(new double[] {-0.005738311176435,-0.010207611670378,0.082662420517928,-0.215733886094368,0.861606487840411,-0.025478530652759,-0.451080697503958})
};
// the following line triggers the exception
EigenDecomposition decomposition =
new EigenDecompositionImpl(mainTridiagonal, secondaryTridiagonal, MathUtils.SAFE_MIN);
double[] eigenValues = decomposition.getRealEigenvalues();
for (int i = 0; i < refEigenValues.length; ++i) {
assertEquals(refEigenValues[i], eigenValues[i], 1.0e-3);
if (refEigenVectors[i].dotProduct(decomposition.getEigenvector(i)) < 0) {
assertEquals(0, refEigenVectors[i].add(decomposition.getEigenvector(i)).getNorm(), 1.0e-5);
} else {
assertEquals(0, refEigenVectors[i].subtract(decomposition.getEigenvector(i)).getNorm(), 1.0e-5);
}
}
} | org.apache.commons.math.linear.EigenDecompositionImplTest::testMathpbx02 | src/test/java/org/apache/commons/math/linear/EigenDecompositionImplTest.java | 188 | src/test/java/org/apache/commons/math/linear/EigenDecompositionImplTest.java | testMathpbx02 | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.math.linear;
import java.util.Arrays;
import java.util.Random;
import org.apache.commons.math.linear.EigenDecomposition;
import org.apache.commons.math.linear.EigenDecompositionImpl;
import org.apache.commons.math.linear.MatrixUtils;
import org.apache.commons.math.linear.RealMatrix;
import org.apache.commons.math.linear.RealVector;
import org.apache.commons.math.linear.TriDiagonalTransformer;
import org.apache.commons.math.util.MathUtils;
import junit.framework.Test;
import junit.framework.TestCase;
import junit.framework.TestSuite;
public class EigenDecompositionImplTest extends TestCase {
private double[] refValues;
private RealMatrix matrix;
public EigenDecompositionImplTest(String name) {
super(name);
}
public static Test suite() {
TestSuite suite = new TestSuite(EigenDecompositionImplTest.class);
suite.setName("EigenDecompositionImpl Tests");
return suite;
}
public void testDimension1() {
RealMatrix matrix =
MatrixUtils.createRealMatrix(new double[][] { { 1.5 } });
EigenDecomposition ed = new EigenDecompositionImpl(matrix, MathUtils.SAFE_MIN);
assertEquals(1.5, ed.getRealEigenvalue(0), 1.0e-15);
}
public void testDimension2() {
RealMatrix matrix =
MatrixUtils.createRealMatrix(new double[][] {
{ 59.0, 12.0 },
{ 12.0, 66.0 }
});
EigenDecomposition ed = new EigenDecompositionImpl(matrix, MathUtils.SAFE_MIN);
assertEquals(75.0, ed.getRealEigenvalue(0), 1.0e-15);
assertEquals(50.0, ed.getRealEigenvalue(1), 1.0e-15);
}
public void testDimension3() {
RealMatrix matrix =
MatrixUtils.createRealMatrix(new double[][] {
{ 39632.0, -4824.0, -16560.0 },
{ -4824.0, 8693.0, 7920.0 },
{ -16560.0, 7920.0, 17300.0 }
});
EigenDecomposition ed = new EigenDecompositionImpl(matrix, MathUtils.SAFE_MIN);
assertEquals(50000.0, ed.getRealEigenvalue(0), 3.0e-11);
assertEquals(12500.0, ed.getRealEigenvalue(1), 3.0e-11);
assertEquals( 3125.0, ed.getRealEigenvalue(2), 3.0e-11);
}
public void testDimension4WithSplit() {
RealMatrix matrix =
MatrixUtils.createRealMatrix(new double[][] {
{ 0.784, -0.288, 0.000, 0.000 },
{ -0.288, 0.616, 0.000, 0.000 },
{ 0.000, 0.000, 0.164, -0.048 },
{ 0.000, 0.000, -0.048, 0.136 }
});
EigenDecomposition ed = new EigenDecompositionImpl(matrix, MathUtils.SAFE_MIN);
assertEquals(1.0, ed.getRealEigenvalue(0), 1.0e-15);
assertEquals(0.4, ed.getRealEigenvalue(1), 1.0e-15);
assertEquals(0.2, ed.getRealEigenvalue(2), 1.0e-15);
assertEquals(0.1, ed.getRealEigenvalue(3), 1.0e-15);
}
public void testDimension4WithoutSplit() {
RealMatrix matrix =
MatrixUtils.createRealMatrix(new double[][] {
{ 0.5608, -0.2016, 0.1152, -0.2976 },
{ -0.2016, 0.4432, -0.2304, 0.1152 },
{ 0.1152, -0.2304, 0.3088, -0.1344 },
{ -0.2976, 0.1152, -0.1344, 0.3872 }
});
EigenDecomposition ed = new EigenDecompositionImpl(matrix, MathUtils.SAFE_MIN);
assertEquals(1.0, ed.getRealEigenvalue(0), 1.0e-15);
assertEquals(0.4, ed.getRealEigenvalue(1), 1.0e-15);
assertEquals(0.2, ed.getRealEigenvalue(2), 1.0e-15);
assertEquals(0.1, ed.getRealEigenvalue(3), 1.0e-15);
}
// the following test triggered an ArrayIndexOutOfBoundsException in commons-math 2.0
public void testMath308() {
double[] mainTridiagonal = {
22.330154644539597, 46.65485522478641, 17.393672330044705, 54.46687435351116, 80.17800767709437
};
double[] secondaryTridiagonal = {
13.04450406501361, -5.977590941539671, 2.9040909856707517, 7.1570352792841225
};
// the reference values have been computed using routine DSTEMR
// from the fortran library LAPACK version 3.2.1
double[] refEigenValues = {
82.044413207204002, 53.456697699894512, 52.536278520113882, 18.847969733754262, 14.138204224043099
};
RealVector[] refEigenVectors = {
new ArrayRealVector(new double[] { -0.000462690386766, -0.002118073109055, 0.011530080757413, 0.252322434584915, 0.967572088232592 }),
new ArrayRealVector(new double[] { 0.314647769490148, 0.750806415553905, -0.167700312025760, -0.537092972407375, 0.143854968127780 }),
new ArrayRealVector(new double[] { 0.222368839324646, 0.514921891363332, -0.021377019336614, 0.801196801016305, -0.207446991247740 }),
new ArrayRealVector(new double[] { 0.713933751051495, -0.190582113553930, 0.671410443368332, -0.056056055955050, 0.006541576993581 }),
new ArrayRealVector(new double[] { 0.584677060845929, -0.367177264979103, -0.721453187784497, 0.052971054621812, -0.005740715188257 })
};
EigenDecomposition decomposition =
new EigenDecompositionImpl(mainTridiagonal, secondaryTridiagonal, MathUtils.SAFE_MIN);
double[] eigenValues = decomposition.getRealEigenvalues();
for (int i = 0; i < refEigenValues.length; ++i) {
assertEquals(refEigenValues[i], eigenValues[i], 1.0e-5);
assertEquals(0, refEigenVectors[i].subtract(decomposition.getEigenvector(i)).getNorm(), 2.0e-7);
}
}
public void testMathpbx02() {
double[] mainTridiagonal = {
7484.860960227216, 18405.28129035345, 13855.225609560746,
10016.708722343366, 559.8117399576674, 6750.190788301587,
71.21428769782159
};
double[] secondaryTridiagonal = {
-4175.088570476366,1975.7955858241994,5193.178422374075,
1995.286659169179,75.34535882933804,-234.0808002076056
};
// the reference values have been computed using routine DSTEMR
// from the fortran library LAPACK version 3.2.1
double[] refEigenValues = {
20654.744890306974412,16828.208208485466457,
6893.155912634994820,6757.083016675340332,
5887.799885688558788,64.309089923240379,
57.992628792736340
};
RealVector[] refEigenVectors = {
new ArrayRealVector(new double[] {-0.270356342026904, 0.852811091326997, 0.399639490702077, 0.198794657813990, 0.019739323307666, 0.000106983022327, -0.000001216636321}),
new ArrayRealVector(new double[] {0.179995273578326,-0.402807848153042,0.701870993525734,0.555058211014888,0.068079148898236,0.000509139115227,-0.000007112235617}),
new ArrayRealVector(new double[] {-0.399582721284727,-0.056629954519333,-0.514406488522827,0.711168164518580,0.225548081276367,0.125943999652923,-0.004321507456014}),
new ArrayRealVector(new double[] {0.058515721572821,0.010200130057739,0.063516274916536,-0.090696087449378,-0.017148420432597,0.991318870265707,-0.034707338554096}),
new ArrayRealVector(new double[] {0.855205995537564,0.327134656629775,-0.265382397060548,0.282690729026706,0.105736068025572,-0.009138126622039,0.000367751821196}),
new ArrayRealVector(new double[] {-0.002913069901144,-0.005177515777101,0.041906334478672,-0.109315918416258,0.436192305456741,0.026307315639535,0.891797507436344}),
new ArrayRealVector(new double[] {-0.005738311176435,-0.010207611670378,0.082662420517928,-0.215733886094368,0.861606487840411,-0.025478530652759,-0.451080697503958})
};
// the following line triggers the exception
EigenDecomposition decomposition =
new EigenDecompositionImpl(mainTridiagonal, secondaryTridiagonal, MathUtils.SAFE_MIN);
double[] eigenValues = decomposition.getRealEigenvalues();
for (int i = 0; i < refEigenValues.length; ++i) {
assertEquals(refEigenValues[i], eigenValues[i], 1.0e-3);
if (refEigenVectors[i].dotProduct(decomposition.getEigenvector(i)) < 0) {
assertEquals(0, refEigenVectors[i].add(decomposition.getEigenvector(i)).getNorm(), 1.0e-5);
} else {
assertEquals(0, refEigenVectors[i].subtract(decomposition.getEigenvector(i)).getNorm(), 1.0e-5);
}
}
}
/** test a matrix already in tridiagonal form. */
public void testTridiagonal() {
Random r = new Random(4366663527842l);
double[] ref = new double[30];
for (int i = 0; i < ref.length; ++i) {
if (i < 5) {
ref[i] = 2 * r.nextDouble() - 1;
} else {
ref[i] = 0.0001 * r.nextDouble() + 6;
}
}
Arrays.sort(ref);
TriDiagonalTransformer t =
new TriDiagonalTransformer(createTestMatrix(r, ref));
EigenDecomposition ed =
new EigenDecompositionImpl(t.getMainDiagonalRef(),
t.getSecondaryDiagonalRef(),
MathUtils.SAFE_MIN);
double[] eigenValues = ed.getRealEigenvalues();
assertEquals(ref.length, eigenValues.length);
for (int i = 0; i < ref.length; ++i) {
assertEquals(ref[ref.length - i - 1], eigenValues[i], 2.0e-14);
}
}
/** test dimensions */
public void testDimensions() {
final int m = matrix.getRowDimension();
EigenDecomposition ed = new EigenDecompositionImpl(matrix, MathUtils.SAFE_MIN);
assertEquals(m, ed.getV().getRowDimension());
assertEquals(m, ed.getV().getColumnDimension());
assertEquals(m, ed.getD().getColumnDimension());
assertEquals(m, ed.getD().getColumnDimension());
assertEquals(m, ed.getVT().getRowDimension());
assertEquals(m, ed.getVT().getColumnDimension());
}
/** test eigenvalues */
public void testEigenvalues() {
EigenDecomposition ed = new EigenDecompositionImpl(matrix, MathUtils.SAFE_MIN);
double[] eigenValues = ed.getRealEigenvalues();
assertEquals(refValues.length, eigenValues.length);
for (int i = 0; i < refValues.length; ++i) {
assertEquals(refValues[i], eigenValues[i], 3.0e-15);
}
}
/** test eigenvalues for a big matrix. */
public void testBigMatrix() {
Random r = new Random(17748333525117l);
double[] bigValues = new double[200];
for (int i = 0; i < bigValues.length; ++i) {
bigValues[i] = 2 * r.nextDouble() - 1;
}
Arrays.sort(bigValues);
EigenDecomposition ed =
new EigenDecompositionImpl(createTestMatrix(r, bigValues), MathUtils.SAFE_MIN);
double[] eigenValues = ed.getRealEigenvalues();
assertEquals(bigValues.length, eigenValues.length);
for (int i = 0; i < bigValues.length; ++i) {
assertEquals(bigValues[bigValues.length - i - 1], eigenValues[i], 2.0e-14);
}
}
/** test eigenvectors */
public void testEigenvectors() {
EigenDecomposition ed = new EigenDecompositionImpl(matrix, MathUtils.SAFE_MIN);
for (int i = 0; i < matrix.getRowDimension(); ++i) {
double lambda = ed.getRealEigenvalue(i);
RealVector v = ed.getEigenvector(i);
RealVector mV = matrix.operate(v);
assertEquals(0, mV.subtract(v.mapMultiplyToSelf(lambda)).getNorm(), 1.0e-13);
}
}
/** test A = VDVt */
public void testAEqualVDVt() {
EigenDecomposition ed = new EigenDecompositionImpl(matrix, MathUtils.SAFE_MIN);
RealMatrix v = ed.getV();
RealMatrix d = ed.getD();
RealMatrix vT = ed.getVT();
double norm = v.multiply(d).multiply(vT).subtract(matrix).getNorm();
assertEquals(0, norm, 6.0e-13);
}
/** test that V is orthogonal */
public void testVOrthogonal() {
RealMatrix v = new EigenDecompositionImpl(matrix, MathUtils.SAFE_MIN).getV();
RealMatrix vTv = v.transpose().multiply(v);
RealMatrix id = MatrixUtils.createRealIdentityMatrix(vTv.getRowDimension());
assertEquals(0, vTv.subtract(id).getNorm(), 2.0e-13);
}
/** test diagonal matrix */
public void testDiagonal() {
double[] diagonal = new double[] { -3.0, -2.0, 2.0, 5.0 };
RealMatrix m = createDiagonalMatrix(diagonal, diagonal.length, diagonal.length);
EigenDecomposition ed = new EigenDecompositionImpl(m, MathUtils.SAFE_MIN);
assertEquals(diagonal[0], ed.getRealEigenvalue(3), 2.0e-15);
assertEquals(diagonal[1], ed.getRealEigenvalue(2), 2.0e-15);
assertEquals(diagonal[2], ed.getRealEigenvalue(1), 2.0e-15);
assertEquals(diagonal[3], ed.getRealEigenvalue(0), 2.0e-15);
}
/**
* Matrix with eigenvalues {8, -1, -1}
*/
public void testRepeatedEigenvalue() {
RealMatrix repeated = MatrixUtils.createRealMatrix(new double[][] {
{3, 2, 4},
{2, 0, 2},
{4, 2, 3}
});
EigenDecomposition ed = new EigenDecompositionImpl(repeated, MathUtils.SAFE_MIN);
checkEigenValues((new double[] {8, -1, -1}), ed, 1E-12);
checkEigenVector((new double[] {2, 1, 2}), ed, 1E-12);
}
/**
* Matrix with eigenvalues {2, 0, 12}
*/
public void testDistinctEigenvalues() {
RealMatrix distinct = MatrixUtils.createRealMatrix(new double[][] {
{3, 1, -4},
{1, 3, -4},
{-4, -4, 8}
});
EigenDecomposition ed = new EigenDecompositionImpl(distinct, MathUtils.SAFE_MIN);
checkEigenValues((new double[] {2, 0, 12}), ed, 1E-12);
checkEigenVector((new double[] {1, -1, 0}), ed, 1E-12);
checkEigenVector((new double[] {1, 1, 1}), ed, 1E-12);
checkEigenVector((new double[] {-1, -1, 2}), ed, 1E-12);
}
/**
* Verifies operation on indefinite matrix
*/
public void testZeroDivide() {
RealMatrix indefinite = MatrixUtils.createRealMatrix(new double [][] {
{ 0.0, 1.0, -1.0 },
{ 1.0, 1.0, 0.0 },
{ -1.0,0.0, 1.0 }
});
EigenDecomposition ed = new EigenDecompositionImpl(indefinite, MathUtils.SAFE_MIN);
checkEigenValues((new double[] {2, 1, -1}), ed, 1E-12);
double isqrt3 = 1/Math.sqrt(3.0);
checkEigenVector((new double[] {isqrt3,isqrt3,-isqrt3}), ed, 1E-12);
double isqrt2 = 1/Math.sqrt(2.0);
checkEigenVector((new double[] {0.0,-isqrt2,-isqrt2}), ed, 1E-12);
double isqrt6 = 1/Math.sqrt(6.0);
checkEigenVector((new double[] {2*isqrt6,-isqrt6,isqrt6}), ed, 1E-12);
}
/**
* Verifies that the given EigenDecomposition has eigenvalues equivalent to
* the targetValues, ignoring the order of the values and allowing
* values to differ by tolerance.
*/
protected void checkEigenValues(double[] targetValues,
EigenDecomposition ed, double tolerance) {
double[] observed = ed.getRealEigenvalues();
for (int i = 0; i < observed.length; i++) {
assertTrue(isIncludedValue(observed[i], targetValues, tolerance));
assertTrue(isIncludedValue(targetValues[i], observed, tolerance));
}
}
/**
* Returns true iff there is an entry within tolerance of value in
* searchArray.
*/
private boolean isIncludedValue(double value, double[] searchArray,
double tolerance) {
boolean found = false;
int i = 0;
while (!found && i < searchArray.length) {
if (Math.abs(value - searchArray[i]) < tolerance) {
found = true;
}
i++;
}
return found;
}
/**
* Returns true iff eigenVector is a scalar multiple of one of the columns
* of ed.getV(). Does not try linear combinations - i.e., should only be
* used to find vectors in one-dimensional eigenspaces.
*/
protected void checkEigenVector(double[] eigenVector,
EigenDecomposition ed, double tolerance) {
assertTrue(isIncludedColumn(eigenVector, ed.getV(), tolerance));
}
/**
* Returns true iff there is a column that is a scalar multiple of column
* in searchMatrix (modulo tolerance)
*/
private boolean isIncludedColumn(double[] column, RealMatrix searchMatrix,
double tolerance) {
boolean found = false;
int i = 0;
while (!found && i < searchMatrix.getColumnDimension()) {
double multiplier = 1.0;
boolean matching = true;
int j = 0;
while (matching && j < searchMatrix.getRowDimension()) {
double colEntry = searchMatrix.getEntry(j, i);
// Use the first entry where both are non-zero as scalar
if (Math.abs(multiplier - 1.0) <= Math.ulp(1.0) && Math.abs(colEntry) > 1E-14
&& Math.abs(column[j]) > 1e-14) {
multiplier = colEntry / column[j];
}
if (Math.abs(column[j] * multiplier - colEntry) > tolerance) {
matching = false;
}
j++;
}
found = matching;
i++;
}
return found;
}
@Override
public void setUp() {
refValues = new double[] {
2.003, 2.002, 2.001, 1.001, 1.000, 0.001
};
matrix = createTestMatrix(new Random(35992629946426l), refValues);
}
@Override
public void tearDown() {
refValues = null;
matrix = null;
}
static RealMatrix createTestMatrix(final Random r, final double[] eigenValues) {
final int n = eigenValues.length;
final RealMatrix v = createOrthogonalMatrix(r, n);
final RealMatrix d = createDiagonalMatrix(eigenValues, n, n);
return v.multiply(d).multiply(v.transpose());
}
public static RealMatrix createOrthogonalMatrix(final Random r, final int size) {
final double[][] data = new double[size][size];
for (int i = 0; i < size; ++i) {
final double[] dataI = data[i];
double norm2 = 0;
do {
// generate randomly row I
for (int j = 0; j < size; ++j) {
dataI[j] = 2 * r.nextDouble() - 1;
}
// project the row in the subspace orthogonal to previous rows
for (int k = 0; k < i; ++k) {
final double[] dataK = data[k];
double dotProduct = 0;
for (int j = 0; j < size; ++j) {
dotProduct += dataI[j] * dataK[j];
}
for (int j = 0; j < size; ++j) {
dataI[j] -= dotProduct * dataK[j];
}
}
// normalize the row
norm2 = 0;
for (final double dataIJ : dataI) {
norm2 += dataIJ * dataIJ;
}
final double inv = 1.0 / Math.sqrt(norm2);
for (int j = 0; j < size; ++j) {
dataI[j] *= inv;
}
} while (norm2 * size < 0.01);
}
return MatrixUtils.createRealMatrix(data);
}
public static RealMatrix createDiagonalMatrix(final double[] diagonal,
final int rows, final int columns) {
final double[][] dData = new double[rows][columns];
for (int i = 0; i < Math.min(rows, columns); ++i) {
dData[i][i] = diagonal[i];
}
return MatrixUtils.createRealMatrix(dData);
}
} | // You are a professional Java test case writer, please create a test case named `testMathpbx02` for the issue `Math-MATH-318`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Math-MATH-318
//
// ## Issue-Title:
// wrong result in eigen decomposition
//
// ## Issue-Description:
//
// Some results computed by EigenDecompositionImpl are wrong. The following case computed by Fortran Lapack fails with version 2.0
//
//
//
//
// ```
// public void testMathpbx02() {
//
// double[] mainTridiagonal = {
// 7484.860960227216, 18405.28129035345, 13855.225609560746,
// 10016.708722343366, 559.8117399576674, 6750.190788301587,
// 71.21428769782159
// };
// double[] secondaryTridiagonal = {
// -4175.088570476366,1975.7955858241994,5193.178422374075,
// 1995.286659169179,75.34535882933804,-234.0808002076056
// };
//
// // the reference values have been computed using routine DSTEMR
// // from the fortran library LAPACK version 3.2.1
// double[] refEigenValues = {
// 20654.744890306974412,16828.208208485466457,
// 6893.155912634994820,6757.083016675340332,
// 5887.799885688558788,64.309089923240379,
// 57.992628792736340
// };
// RealVector[] refEigenVectors = {
// new ArrayRealVector(new double[] {-0.270356342026904, 0.852811091326997, 0.399639490702077, 0.198794657813990, 0.019739323307666, 0.000106983022327, -0.000001216636321}),
// new ArrayRealVector(new double[] {0.179995273578326,-0.402807848153042,0.701870993525734,0.555058211014888,0.068079148898236,0.000509139115227,-0.000007112235617}),
// new ArrayRealVector(new double[] {-0.399582721284727,-0.056629954519333,-0.514406488522827,0.711168164518580,0.225548081276367,0.125943999652923,-0.004321507456014}),
// new ArrayRealVector(new double[] {0.058515721572821,0.010200130057739,0.063516274916536,-0.090696087449378,-0.017148420432597,0.991318870265707,-0.034707338554096}),
// new ArrayRealVector(new double[] {0.855205995537564,0.327134656629775,-0.265382397060548,0.282690729026706,0.105736068025572,-0.009138126622039,0.000367751821196}),
// new ArrayRealVector(new double[] {-0.002913069901144,-0.005177515777101,0.041906334478672,-0.109315918416258,0.436192305456741,0.026307315639535,0.89
public void testMathpbx02() {
| 188 | 80 | 144 | src/test/java/org/apache/commons/math/linear/EigenDecompositionImplTest.java | src/test/java | ```markdown
## Issue-ID: Math-MATH-318
## Issue-Title:
wrong result in eigen decomposition
## Issue-Description:
Some results computed by EigenDecompositionImpl are wrong. The following case computed by Fortran Lapack fails with version 2.0
```
public void testMathpbx02() {
double[] mainTridiagonal = {
7484.860960227216, 18405.28129035345, 13855.225609560746,
10016.708722343366, 559.8117399576674, 6750.190788301587,
71.21428769782159
};
double[] secondaryTridiagonal = {
-4175.088570476366,1975.7955858241994,5193.178422374075,
1995.286659169179,75.34535882933804,-234.0808002076056
};
// the reference values have been computed using routine DSTEMR
// from the fortran library LAPACK version 3.2.1
double[] refEigenValues = {
20654.744890306974412,16828.208208485466457,
6893.155912634994820,6757.083016675340332,
5887.799885688558788,64.309089923240379,
57.992628792736340
};
RealVector[] refEigenVectors = {
new ArrayRealVector(new double[] {-0.270356342026904, 0.852811091326997, 0.399639490702077, 0.198794657813990, 0.019739323307666, 0.000106983022327, -0.000001216636321}),
new ArrayRealVector(new double[] {0.179995273578326,-0.402807848153042,0.701870993525734,0.555058211014888,0.068079148898236,0.000509139115227,-0.000007112235617}),
new ArrayRealVector(new double[] {-0.399582721284727,-0.056629954519333,-0.514406488522827,0.711168164518580,0.225548081276367,0.125943999652923,-0.004321507456014}),
new ArrayRealVector(new double[] {0.058515721572821,0.010200130057739,0.063516274916536,-0.090696087449378,-0.017148420432597,0.991318870265707,-0.034707338554096}),
new ArrayRealVector(new double[] {0.855205995537564,0.327134656629775,-0.265382397060548,0.282690729026706,0.105736068025572,-0.009138126622039,0.000367751821196}),
new ArrayRealVector(new double[] {-0.002913069901144,-0.005177515777101,0.041906334478672,-0.109315918416258,0.436192305456741,0.026307315639535,0.89
```
You are a professional Java test case writer, please create a test case named `testMathpbx02` for the issue `Math-MATH-318`, utilizing the provided issue report information and the following function signature.
```java
public void testMathpbx02() {
```
| 144 | [
"org.apache.commons.math.linear.EigenDecompositionImpl"
] | 79a2711195f47bf12735df29374553ae6e7130d66000fe59d2565e7bf4dc8bc9 | public void testMathpbx02() | // You are a professional Java test case writer, please create a test case named `testMathpbx02` for the issue `Math-MATH-318`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Math-MATH-318
//
// ## Issue-Title:
// wrong result in eigen decomposition
//
// ## Issue-Description:
//
// Some results computed by EigenDecompositionImpl are wrong. The following case computed by Fortran Lapack fails with version 2.0
//
//
//
//
// ```
// public void testMathpbx02() {
//
// double[] mainTridiagonal = {
// 7484.860960227216, 18405.28129035345, 13855.225609560746,
// 10016.708722343366, 559.8117399576674, 6750.190788301587,
// 71.21428769782159
// };
// double[] secondaryTridiagonal = {
// -4175.088570476366,1975.7955858241994,5193.178422374075,
// 1995.286659169179,75.34535882933804,-234.0808002076056
// };
//
// // the reference values have been computed using routine DSTEMR
// // from the fortran library LAPACK version 3.2.1
// double[] refEigenValues = {
// 20654.744890306974412,16828.208208485466457,
// 6893.155912634994820,6757.083016675340332,
// 5887.799885688558788,64.309089923240379,
// 57.992628792736340
// };
// RealVector[] refEigenVectors = {
// new ArrayRealVector(new double[] {-0.270356342026904, 0.852811091326997, 0.399639490702077, 0.198794657813990, 0.019739323307666, 0.000106983022327, -0.000001216636321}),
// new ArrayRealVector(new double[] {0.179995273578326,-0.402807848153042,0.701870993525734,0.555058211014888,0.068079148898236,0.000509139115227,-0.000007112235617}),
// new ArrayRealVector(new double[] {-0.399582721284727,-0.056629954519333,-0.514406488522827,0.711168164518580,0.225548081276367,0.125943999652923,-0.004321507456014}),
// new ArrayRealVector(new double[] {0.058515721572821,0.010200130057739,0.063516274916536,-0.090696087449378,-0.017148420432597,0.991318870265707,-0.034707338554096}),
// new ArrayRealVector(new double[] {0.855205995537564,0.327134656629775,-0.265382397060548,0.282690729026706,0.105736068025572,-0.009138126622039,0.000367751821196}),
// new ArrayRealVector(new double[] {-0.002913069901144,-0.005177515777101,0.041906334478672,-0.109315918416258,0.436192305456741,0.026307315639535,0.89
| Math | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.math.linear;
import java.util.Arrays;
import java.util.Random;
import org.apache.commons.math.linear.EigenDecomposition;
import org.apache.commons.math.linear.EigenDecompositionImpl;
import org.apache.commons.math.linear.MatrixUtils;
import org.apache.commons.math.linear.RealMatrix;
import org.apache.commons.math.linear.RealVector;
import org.apache.commons.math.linear.TriDiagonalTransformer;
import org.apache.commons.math.util.MathUtils;
import junit.framework.Test;
import junit.framework.TestCase;
import junit.framework.TestSuite;
public class EigenDecompositionImplTest extends TestCase {
private double[] refValues;
private RealMatrix matrix;
public EigenDecompositionImplTest(String name) {
super(name);
}
public static Test suite() {
TestSuite suite = new TestSuite(EigenDecompositionImplTest.class);
suite.setName("EigenDecompositionImpl Tests");
return suite;
}
public void testDimension1() {
RealMatrix matrix =
MatrixUtils.createRealMatrix(new double[][] { { 1.5 } });
EigenDecomposition ed = new EigenDecompositionImpl(matrix, MathUtils.SAFE_MIN);
assertEquals(1.5, ed.getRealEigenvalue(0), 1.0e-15);
}
public void testDimension2() {
RealMatrix matrix =
MatrixUtils.createRealMatrix(new double[][] {
{ 59.0, 12.0 },
{ 12.0, 66.0 }
});
EigenDecomposition ed = new EigenDecompositionImpl(matrix, MathUtils.SAFE_MIN);
assertEquals(75.0, ed.getRealEigenvalue(0), 1.0e-15);
assertEquals(50.0, ed.getRealEigenvalue(1), 1.0e-15);
}
public void testDimension3() {
RealMatrix matrix =
MatrixUtils.createRealMatrix(new double[][] {
{ 39632.0, -4824.0, -16560.0 },
{ -4824.0, 8693.0, 7920.0 },
{ -16560.0, 7920.0, 17300.0 }
});
EigenDecomposition ed = new EigenDecompositionImpl(matrix, MathUtils.SAFE_MIN);
assertEquals(50000.0, ed.getRealEigenvalue(0), 3.0e-11);
assertEquals(12500.0, ed.getRealEigenvalue(1), 3.0e-11);
assertEquals( 3125.0, ed.getRealEigenvalue(2), 3.0e-11);
}
public void testDimension4WithSplit() {
RealMatrix matrix =
MatrixUtils.createRealMatrix(new double[][] {
{ 0.784, -0.288, 0.000, 0.000 },
{ -0.288, 0.616, 0.000, 0.000 },
{ 0.000, 0.000, 0.164, -0.048 },
{ 0.000, 0.000, -0.048, 0.136 }
});
EigenDecomposition ed = new EigenDecompositionImpl(matrix, MathUtils.SAFE_MIN);
assertEquals(1.0, ed.getRealEigenvalue(0), 1.0e-15);
assertEquals(0.4, ed.getRealEigenvalue(1), 1.0e-15);
assertEquals(0.2, ed.getRealEigenvalue(2), 1.0e-15);
assertEquals(0.1, ed.getRealEigenvalue(3), 1.0e-15);
}
public void testDimension4WithoutSplit() {
RealMatrix matrix =
MatrixUtils.createRealMatrix(new double[][] {
{ 0.5608, -0.2016, 0.1152, -0.2976 },
{ -0.2016, 0.4432, -0.2304, 0.1152 },
{ 0.1152, -0.2304, 0.3088, -0.1344 },
{ -0.2976, 0.1152, -0.1344, 0.3872 }
});
EigenDecomposition ed = new EigenDecompositionImpl(matrix, MathUtils.SAFE_MIN);
assertEquals(1.0, ed.getRealEigenvalue(0), 1.0e-15);
assertEquals(0.4, ed.getRealEigenvalue(1), 1.0e-15);
assertEquals(0.2, ed.getRealEigenvalue(2), 1.0e-15);
assertEquals(0.1, ed.getRealEigenvalue(3), 1.0e-15);
}
// the following test triggered an ArrayIndexOutOfBoundsException in commons-math 2.0
public void testMath308() {
double[] mainTridiagonal = {
22.330154644539597, 46.65485522478641, 17.393672330044705, 54.46687435351116, 80.17800767709437
};
double[] secondaryTridiagonal = {
13.04450406501361, -5.977590941539671, 2.9040909856707517, 7.1570352792841225
};
// the reference values have been computed using routine DSTEMR
// from the fortran library LAPACK version 3.2.1
double[] refEigenValues = {
82.044413207204002, 53.456697699894512, 52.536278520113882, 18.847969733754262, 14.138204224043099
};
RealVector[] refEigenVectors = {
new ArrayRealVector(new double[] { -0.000462690386766, -0.002118073109055, 0.011530080757413, 0.252322434584915, 0.967572088232592 }),
new ArrayRealVector(new double[] { 0.314647769490148, 0.750806415553905, -0.167700312025760, -0.537092972407375, 0.143854968127780 }),
new ArrayRealVector(new double[] { 0.222368839324646, 0.514921891363332, -0.021377019336614, 0.801196801016305, -0.207446991247740 }),
new ArrayRealVector(new double[] { 0.713933751051495, -0.190582113553930, 0.671410443368332, -0.056056055955050, 0.006541576993581 }),
new ArrayRealVector(new double[] { 0.584677060845929, -0.367177264979103, -0.721453187784497, 0.052971054621812, -0.005740715188257 })
};
EigenDecomposition decomposition =
new EigenDecompositionImpl(mainTridiagonal, secondaryTridiagonal, MathUtils.SAFE_MIN);
double[] eigenValues = decomposition.getRealEigenvalues();
for (int i = 0; i < refEigenValues.length; ++i) {
assertEquals(refEigenValues[i], eigenValues[i], 1.0e-5);
assertEquals(0, refEigenVectors[i].subtract(decomposition.getEigenvector(i)).getNorm(), 2.0e-7);
}
}
public void testMathpbx02() {
double[] mainTridiagonal = {
7484.860960227216, 18405.28129035345, 13855.225609560746,
10016.708722343366, 559.8117399576674, 6750.190788301587,
71.21428769782159
};
double[] secondaryTridiagonal = {
-4175.088570476366,1975.7955858241994,5193.178422374075,
1995.286659169179,75.34535882933804,-234.0808002076056
};
// the reference values have been computed using routine DSTEMR
// from the fortran library LAPACK version 3.2.1
double[] refEigenValues = {
20654.744890306974412,16828.208208485466457,
6893.155912634994820,6757.083016675340332,
5887.799885688558788,64.309089923240379,
57.992628792736340
};
RealVector[] refEigenVectors = {
new ArrayRealVector(new double[] {-0.270356342026904, 0.852811091326997, 0.399639490702077, 0.198794657813990, 0.019739323307666, 0.000106983022327, -0.000001216636321}),
new ArrayRealVector(new double[] {0.179995273578326,-0.402807848153042,0.701870993525734,0.555058211014888,0.068079148898236,0.000509139115227,-0.000007112235617}),
new ArrayRealVector(new double[] {-0.399582721284727,-0.056629954519333,-0.514406488522827,0.711168164518580,0.225548081276367,0.125943999652923,-0.004321507456014}),
new ArrayRealVector(new double[] {0.058515721572821,0.010200130057739,0.063516274916536,-0.090696087449378,-0.017148420432597,0.991318870265707,-0.034707338554096}),
new ArrayRealVector(new double[] {0.855205995537564,0.327134656629775,-0.265382397060548,0.282690729026706,0.105736068025572,-0.009138126622039,0.000367751821196}),
new ArrayRealVector(new double[] {-0.002913069901144,-0.005177515777101,0.041906334478672,-0.109315918416258,0.436192305456741,0.026307315639535,0.891797507436344}),
new ArrayRealVector(new double[] {-0.005738311176435,-0.010207611670378,0.082662420517928,-0.215733886094368,0.861606487840411,-0.025478530652759,-0.451080697503958})
};
// the following line triggers the exception
EigenDecomposition decomposition =
new EigenDecompositionImpl(mainTridiagonal, secondaryTridiagonal, MathUtils.SAFE_MIN);
double[] eigenValues = decomposition.getRealEigenvalues();
for (int i = 0; i < refEigenValues.length; ++i) {
assertEquals(refEigenValues[i], eigenValues[i], 1.0e-3);
if (refEigenVectors[i].dotProduct(decomposition.getEigenvector(i)) < 0) {
assertEquals(0, refEigenVectors[i].add(decomposition.getEigenvector(i)).getNorm(), 1.0e-5);
} else {
assertEquals(0, refEigenVectors[i].subtract(decomposition.getEigenvector(i)).getNorm(), 1.0e-5);
}
}
}
/** test a matrix already in tridiagonal form. */
public void testTridiagonal() {
Random r = new Random(4366663527842l);
double[] ref = new double[30];
for (int i = 0; i < ref.length; ++i) {
if (i < 5) {
ref[i] = 2 * r.nextDouble() - 1;
} else {
ref[i] = 0.0001 * r.nextDouble() + 6;
}
}
Arrays.sort(ref);
TriDiagonalTransformer t =
new TriDiagonalTransformer(createTestMatrix(r, ref));
EigenDecomposition ed =
new EigenDecompositionImpl(t.getMainDiagonalRef(),
t.getSecondaryDiagonalRef(),
MathUtils.SAFE_MIN);
double[] eigenValues = ed.getRealEigenvalues();
assertEquals(ref.length, eigenValues.length);
for (int i = 0; i < ref.length; ++i) {
assertEquals(ref[ref.length - i - 1], eigenValues[i], 2.0e-14);
}
}
/** test dimensions */
public void testDimensions() {
final int m = matrix.getRowDimension();
EigenDecomposition ed = new EigenDecompositionImpl(matrix, MathUtils.SAFE_MIN);
assertEquals(m, ed.getV().getRowDimension());
assertEquals(m, ed.getV().getColumnDimension());
assertEquals(m, ed.getD().getColumnDimension());
assertEquals(m, ed.getD().getColumnDimension());
assertEquals(m, ed.getVT().getRowDimension());
assertEquals(m, ed.getVT().getColumnDimension());
}
/** test eigenvalues */
public void testEigenvalues() {
EigenDecomposition ed = new EigenDecompositionImpl(matrix, MathUtils.SAFE_MIN);
double[] eigenValues = ed.getRealEigenvalues();
assertEquals(refValues.length, eigenValues.length);
for (int i = 0; i < refValues.length; ++i) {
assertEquals(refValues[i], eigenValues[i], 3.0e-15);
}
}
/** test eigenvalues for a big matrix. */
public void testBigMatrix() {
Random r = new Random(17748333525117l);
double[] bigValues = new double[200];
for (int i = 0; i < bigValues.length; ++i) {
bigValues[i] = 2 * r.nextDouble() - 1;
}
Arrays.sort(bigValues);
EigenDecomposition ed =
new EigenDecompositionImpl(createTestMatrix(r, bigValues), MathUtils.SAFE_MIN);
double[] eigenValues = ed.getRealEigenvalues();
assertEquals(bigValues.length, eigenValues.length);
for (int i = 0; i < bigValues.length; ++i) {
assertEquals(bigValues[bigValues.length - i - 1], eigenValues[i], 2.0e-14);
}
}
/** test eigenvectors */
public void testEigenvectors() {
EigenDecomposition ed = new EigenDecompositionImpl(matrix, MathUtils.SAFE_MIN);
for (int i = 0; i < matrix.getRowDimension(); ++i) {
double lambda = ed.getRealEigenvalue(i);
RealVector v = ed.getEigenvector(i);
RealVector mV = matrix.operate(v);
assertEquals(0, mV.subtract(v.mapMultiplyToSelf(lambda)).getNorm(), 1.0e-13);
}
}
/** test A = VDVt */
public void testAEqualVDVt() {
EigenDecomposition ed = new EigenDecompositionImpl(matrix, MathUtils.SAFE_MIN);
RealMatrix v = ed.getV();
RealMatrix d = ed.getD();
RealMatrix vT = ed.getVT();
double norm = v.multiply(d).multiply(vT).subtract(matrix).getNorm();
assertEquals(0, norm, 6.0e-13);
}
/** test that V is orthogonal */
public void testVOrthogonal() {
RealMatrix v = new EigenDecompositionImpl(matrix, MathUtils.SAFE_MIN).getV();
RealMatrix vTv = v.transpose().multiply(v);
RealMatrix id = MatrixUtils.createRealIdentityMatrix(vTv.getRowDimension());
assertEquals(0, vTv.subtract(id).getNorm(), 2.0e-13);
}
/** test diagonal matrix */
public void testDiagonal() {
double[] diagonal = new double[] { -3.0, -2.0, 2.0, 5.0 };
RealMatrix m = createDiagonalMatrix(diagonal, diagonal.length, diagonal.length);
EigenDecomposition ed = new EigenDecompositionImpl(m, MathUtils.SAFE_MIN);
assertEquals(diagonal[0], ed.getRealEigenvalue(3), 2.0e-15);
assertEquals(diagonal[1], ed.getRealEigenvalue(2), 2.0e-15);
assertEquals(diagonal[2], ed.getRealEigenvalue(1), 2.0e-15);
assertEquals(diagonal[3], ed.getRealEigenvalue(0), 2.0e-15);
}
/**
* Matrix with eigenvalues {8, -1, -1}
*/
public void testRepeatedEigenvalue() {
RealMatrix repeated = MatrixUtils.createRealMatrix(new double[][] {
{3, 2, 4},
{2, 0, 2},
{4, 2, 3}
});
EigenDecomposition ed = new EigenDecompositionImpl(repeated, MathUtils.SAFE_MIN);
checkEigenValues((new double[] {8, -1, -1}), ed, 1E-12);
checkEigenVector((new double[] {2, 1, 2}), ed, 1E-12);
}
/**
* Matrix with eigenvalues {2, 0, 12}
*/
public void testDistinctEigenvalues() {
RealMatrix distinct = MatrixUtils.createRealMatrix(new double[][] {
{3, 1, -4},
{1, 3, -4},
{-4, -4, 8}
});
EigenDecomposition ed = new EigenDecompositionImpl(distinct, MathUtils.SAFE_MIN);
checkEigenValues((new double[] {2, 0, 12}), ed, 1E-12);
checkEigenVector((new double[] {1, -1, 0}), ed, 1E-12);
checkEigenVector((new double[] {1, 1, 1}), ed, 1E-12);
checkEigenVector((new double[] {-1, -1, 2}), ed, 1E-12);
}
/**
* Verifies operation on indefinite matrix
*/
public void testZeroDivide() {
RealMatrix indefinite = MatrixUtils.createRealMatrix(new double [][] {
{ 0.0, 1.0, -1.0 },
{ 1.0, 1.0, 0.0 },
{ -1.0,0.0, 1.0 }
});
EigenDecomposition ed = new EigenDecompositionImpl(indefinite, MathUtils.SAFE_MIN);
checkEigenValues((new double[] {2, 1, -1}), ed, 1E-12);
double isqrt3 = 1/Math.sqrt(3.0);
checkEigenVector((new double[] {isqrt3,isqrt3,-isqrt3}), ed, 1E-12);
double isqrt2 = 1/Math.sqrt(2.0);
checkEigenVector((new double[] {0.0,-isqrt2,-isqrt2}), ed, 1E-12);
double isqrt6 = 1/Math.sqrt(6.0);
checkEigenVector((new double[] {2*isqrt6,-isqrt6,isqrt6}), ed, 1E-12);
}
/**
* Verifies that the given EigenDecomposition has eigenvalues equivalent to
* the targetValues, ignoring the order of the values and allowing
* values to differ by tolerance.
*/
protected void checkEigenValues(double[] targetValues,
EigenDecomposition ed, double tolerance) {
double[] observed = ed.getRealEigenvalues();
for (int i = 0; i < observed.length; i++) {
assertTrue(isIncludedValue(observed[i], targetValues, tolerance));
assertTrue(isIncludedValue(targetValues[i], observed, tolerance));
}
}
/**
* Returns true iff there is an entry within tolerance of value in
* searchArray.
*/
private boolean isIncludedValue(double value, double[] searchArray,
double tolerance) {
boolean found = false;
int i = 0;
while (!found && i < searchArray.length) {
if (Math.abs(value - searchArray[i]) < tolerance) {
found = true;
}
i++;
}
return found;
}
/**
* Returns true iff eigenVector is a scalar multiple of one of the columns
* of ed.getV(). Does not try linear combinations - i.e., should only be
* used to find vectors in one-dimensional eigenspaces.
*/
protected void checkEigenVector(double[] eigenVector,
EigenDecomposition ed, double tolerance) {
assertTrue(isIncludedColumn(eigenVector, ed.getV(), tolerance));
}
/**
* Returns true iff there is a column that is a scalar multiple of column
* in searchMatrix (modulo tolerance)
*/
private boolean isIncludedColumn(double[] column, RealMatrix searchMatrix,
double tolerance) {
boolean found = false;
int i = 0;
while (!found && i < searchMatrix.getColumnDimension()) {
double multiplier = 1.0;
boolean matching = true;
int j = 0;
while (matching && j < searchMatrix.getRowDimension()) {
double colEntry = searchMatrix.getEntry(j, i);
// Use the first entry where both are non-zero as scalar
if (Math.abs(multiplier - 1.0) <= Math.ulp(1.0) && Math.abs(colEntry) > 1E-14
&& Math.abs(column[j]) > 1e-14) {
multiplier = colEntry / column[j];
}
if (Math.abs(column[j] * multiplier - colEntry) > tolerance) {
matching = false;
}
j++;
}
found = matching;
i++;
}
return found;
}
@Override
public void setUp() {
refValues = new double[] {
2.003, 2.002, 2.001, 1.001, 1.000, 0.001
};
matrix = createTestMatrix(new Random(35992629946426l), refValues);
}
@Override
public void tearDown() {
refValues = null;
matrix = null;
}
static RealMatrix createTestMatrix(final Random r, final double[] eigenValues) {
final int n = eigenValues.length;
final RealMatrix v = createOrthogonalMatrix(r, n);
final RealMatrix d = createDiagonalMatrix(eigenValues, n, n);
return v.multiply(d).multiply(v.transpose());
}
public static RealMatrix createOrthogonalMatrix(final Random r, final int size) {
final double[][] data = new double[size][size];
for (int i = 0; i < size; ++i) {
final double[] dataI = data[i];
double norm2 = 0;
do {
// generate randomly row I
for (int j = 0; j < size; ++j) {
dataI[j] = 2 * r.nextDouble() - 1;
}
// project the row in the subspace orthogonal to previous rows
for (int k = 0; k < i; ++k) {
final double[] dataK = data[k];
double dotProduct = 0;
for (int j = 0; j < size; ++j) {
dotProduct += dataI[j] * dataK[j];
}
for (int j = 0; j < size; ++j) {
dataI[j] -= dotProduct * dataK[j];
}
}
// normalize the row
norm2 = 0;
for (final double dataIJ : dataI) {
norm2 += dataIJ * dataIJ;
}
final double inv = 1.0 / Math.sqrt(norm2);
for (int j = 0; j < size; ++j) {
dataI[j] *= inv;
}
} while (norm2 * size < 0.01);
}
return MatrixUtils.createRealMatrix(data);
}
public static RealMatrix createDiagonalMatrix(final double[] diagonal,
final int rows, final int columns) {
final double[][] dData = new double[rows][columns];
for (int i = 0; i < Math.min(rows, columns); ++i) {
dData[i][i] = diagonal[i];
}
return MatrixUtils.createRealMatrix(dData);
}
} |
||
public void testSimple() throws Exception {
final ObjectMapper mapper = new ObjectMapper();
final String json = aposToQuotes("{'schemas': [{\n"
+ " 'name': 'FoodMart'\n"
+ "}]}\n");
mapper.readValue(json, JsonRoot.class);
} | com.fasterxml.jackson.databind.objectid.Objecid1083Test::testSimple | src/test/java/com/fasterxml/jackson/databind/objectid/Objecid1083Test.java | 43 | src/test/java/com/fasterxml/jackson/databind/objectid/Objecid1083Test.java | testSimple | package com.fasterxml.jackson.databind.objectid;
import java.util.*;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.core.*;
import com.fasterxml.jackson.databind.*;
public class Objecid1083Test extends BaseMapTest
{
public static class JsonRoot {
public final List<JsonSchema> schemas = new ArrayList<JsonSchema>();
}
@JsonTypeInfo(
use = JsonTypeInfo.Id.NAME,
property = "type",
defaultImpl = JsonMapSchema.class)
@JsonSubTypes({
@JsonSubTypes.Type(value = JsonMapSchema.class, name = "map"),
@JsonSubTypes.Type(value = JsonJdbcSchema.class, name = "jdbc") })
public static abstract class JsonSchema {
public String name;
}
static class JsonMapSchema extends JsonSchema { }
static class JsonJdbcSchema extends JsonSchema { }
/*
/*****************************************************
/* Unit tests, external id deserialization
/*****************************************************
*/
public void testSimple() throws Exception {
final ObjectMapper mapper = new ObjectMapper();
final String json = aposToQuotes("{'schemas': [{\n"
+ " 'name': 'FoodMart'\n"
+ "}]}\n");
mapper.readValue(json, JsonRoot.class);
}
} | // You are a professional Java test case writer, please create a test case named `testSimple` for the issue `JacksonDatabind-1083`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonDatabind-1083
//
// ## Issue-Title:
// Field in base class is not recognized, when using @JsonType.defaultImpl
//
// ## Issue-Description:
// When deserializing JSON to Java POJOS, a field inherited from a base class is not recognized. Here is the stack:
//
//
//
// ```
// com.fasterxml.jackson.databind.exc.UnrecognizedPropertyException: Unrecognized field "name" (class org.apache.calcite.model.JsonMapSchema), not marked as ignorable (2 known properties: "functions", "tables"])
// at [Source: {
// version: '1.0',
// schemas: [
// {
// name: 'FoodMart',
// tables: [
// {
// name: 'time_by_day',
// columns: [
// {
// name: 'time_id'
// }
// ]
// },
// {
// name: 'sales_fact_1997',
// columns: [
// {
// name: 'time_id'
// }
// ]
// }
// ]
// }
// ]
// }; line: 24, column: 7] (through reference chain: org.apache.calcite.model.JsonRoot["schemas"]->java.util.ArrayList[0]->org.apache.calcite.model.JsonMapSchema["name"])
//
// at com.fasterxml.jackson.databind.exc.UnrecognizedPropertyException.from(UnrecognizedPropertyException.java:62)
// at com.fasterxml.jackson.databind.DeserializationContext.reportUnknownProperty(DeserializationContext.java:855)
// at com.fasterxml.jackson.databind.deser.std.StdDeserializer.handleUnknownProperty(StdDeserializer.java:1083)
// at com.fasterxml.jackson.databind.deser.BeanDeserializerBase.handleUnknownProperty(BeanDeserializerBase.java:1389)
// at com.fasterxml.jackson.databind.deser.BeanDeserializerBase.handleUnknownVanilla(BeanDeserializerBase.java:1367)
// at com.fasterxml.jackson.databind.deser.BeanDeserializer.vanillaDeserialize(BeanDeserializer.java:266)
// at com.fasterxml.jackson.databind.deser.BeanDeserializer._deserializeOther(BeanDeserializer.java:163)
// at com.fasterxml.jackson.databind.deser.BeanDeserializer.deserialize(BeanDeserializer.java:135)
// at com.fasterxml.jackson.databind.jsontype.impl.AsPropertyTypeDeserializer._deserializeTypedUsingDefaultImpl(AsPropertyTypeDeserializer.java:136)
// at com.fasterxml.jackson.databind.jsontype.impl.AsPropertyTypeDeserializer.deserializeTypedFromObject(AsPropertyTypeDeserializer.java:99)
// at com.fasterxml.jackson.databind.deser.AbstractDeserializer.deserializeWithType(AbstractDeserializer.java:142)
// at com.fasterxml.jackson.databind.deser.std.CollectionDeserializer.deserialize(CollectionDeserializer.java:
public void testSimple() throws Exception {
| 43 | /*
/*****************************************************
/* Unit tests, external id deserialization
/*****************************************************
*/ | 37 | 37 | src/test/java/com/fasterxml/jackson/databind/objectid/Objecid1083Test.java | src/test/java | ```markdown
## Issue-ID: JacksonDatabind-1083
## Issue-Title:
Field in base class is not recognized, when using @JsonType.defaultImpl
## Issue-Description:
When deserializing JSON to Java POJOS, a field inherited from a base class is not recognized. Here is the stack:
```
com.fasterxml.jackson.databind.exc.UnrecognizedPropertyException: Unrecognized field "name" (class org.apache.calcite.model.JsonMapSchema), not marked as ignorable (2 known properties: "functions", "tables"])
at [Source: {
version: '1.0',
schemas: [
{
name: 'FoodMart',
tables: [
{
name: 'time_by_day',
columns: [
{
name: 'time_id'
}
]
},
{
name: 'sales_fact_1997',
columns: [
{
name: 'time_id'
}
]
}
]
}
]
}; line: 24, column: 7] (through reference chain: org.apache.calcite.model.JsonRoot["schemas"]->java.util.ArrayList[0]->org.apache.calcite.model.JsonMapSchema["name"])
at com.fasterxml.jackson.databind.exc.UnrecognizedPropertyException.from(UnrecognizedPropertyException.java:62)
at com.fasterxml.jackson.databind.DeserializationContext.reportUnknownProperty(DeserializationContext.java:855)
at com.fasterxml.jackson.databind.deser.std.StdDeserializer.handleUnknownProperty(StdDeserializer.java:1083)
at com.fasterxml.jackson.databind.deser.BeanDeserializerBase.handleUnknownProperty(BeanDeserializerBase.java:1389)
at com.fasterxml.jackson.databind.deser.BeanDeserializerBase.handleUnknownVanilla(BeanDeserializerBase.java:1367)
at com.fasterxml.jackson.databind.deser.BeanDeserializer.vanillaDeserialize(BeanDeserializer.java:266)
at com.fasterxml.jackson.databind.deser.BeanDeserializer._deserializeOther(BeanDeserializer.java:163)
at com.fasterxml.jackson.databind.deser.BeanDeserializer.deserialize(BeanDeserializer.java:135)
at com.fasterxml.jackson.databind.jsontype.impl.AsPropertyTypeDeserializer._deserializeTypedUsingDefaultImpl(AsPropertyTypeDeserializer.java:136)
at com.fasterxml.jackson.databind.jsontype.impl.AsPropertyTypeDeserializer.deserializeTypedFromObject(AsPropertyTypeDeserializer.java:99)
at com.fasterxml.jackson.databind.deser.AbstractDeserializer.deserializeWithType(AbstractDeserializer.java:142)
at com.fasterxml.jackson.databind.deser.std.CollectionDeserializer.deserialize(CollectionDeserializer.java:
```
You are a professional Java test case writer, please create a test case named `testSimple` for the issue `JacksonDatabind-1083`, utilizing the provided issue report information and the following function signature.
```java
public void testSimple() throws Exception {
```
| 37 | [
"com.fasterxml.jackson.databind.type.SimpleType"
] | 7ab1bdb2549a1c95c52fab025190831402080f55ff044590ef1f4f84bf7d7655 | public void testSimple() throws Exception | // You are a professional Java test case writer, please create a test case named `testSimple` for the issue `JacksonDatabind-1083`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonDatabind-1083
//
// ## Issue-Title:
// Field in base class is not recognized, when using @JsonType.defaultImpl
//
// ## Issue-Description:
// When deserializing JSON to Java POJOS, a field inherited from a base class is not recognized. Here is the stack:
//
//
//
// ```
// com.fasterxml.jackson.databind.exc.UnrecognizedPropertyException: Unrecognized field "name" (class org.apache.calcite.model.JsonMapSchema), not marked as ignorable (2 known properties: "functions", "tables"])
// at [Source: {
// version: '1.0',
// schemas: [
// {
// name: 'FoodMart',
// tables: [
// {
// name: 'time_by_day',
// columns: [
// {
// name: 'time_id'
// }
// ]
// },
// {
// name: 'sales_fact_1997',
// columns: [
// {
// name: 'time_id'
// }
// ]
// }
// ]
// }
// ]
// }; line: 24, column: 7] (through reference chain: org.apache.calcite.model.JsonRoot["schemas"]->java.util.ArrayList[0]->org.apache.calcite.model.JsonMapSchema["name"])
//
// at com.fasterxml.jackson.databind.exc.UnrecognizedPropertyException.from(UnrecognizedPropertyException.java:62)
// at com.fasterxml.jackson.databind.DeserializationContext.reportUnknownProperty(DeserializationContext.java:855)
// at com.fasterxml.jackson.databind.deser.std.StdDeserializer.handleUnknownProperty(StdDeserializer.java:1083)
// at com.fasterxml.jackson.databind.deser.BeanDeserializerBase.handleUnknownProperty(BeanDeserializerBase.java:1389)
// at com.fasterxml.jackson.databind.deser.BeanDeserializerBase.handleUnknownVanilla(BeanDeserializerBase.java:1367)
// at com.fasterxml.jackson.databind.deser.BeanDeserializer.vanillaDeserialize(BeanDeserializer.java:266)
// at com.fasterxml.jackson.databind.deser.BeanDeserializer._deserializeOther(BeanDeserializer.java:163)
// at com.fasterxml.jackson.databind.deser.BeanDeserializer.deserialize(BeanDeserializer.java:135)
// at com.fasterxml.jackson.databind.jsontype.impl.AsPropertyTypeDeserializer._deserializeTypedUsingDefaultImpl(AsPropertyTypeDeserializer.java:136)
// at com.fasterxml.jackson.databind.jsontype.impl.AsPropertyTypeDeserializer.deserializeTypedFromObject(AsPropertyTypeDeserializer.java:99)
// at com.fasterxml.jackson.databind.deser.AbstractDeserializer.deserializeWithType(AbstractDeserializer.java:142)
// at com.fasterxml.jackson.databind.deser.std.CollectionDeserializer.deserialize(CollectionDeserializer.java:
| JacksonDatabind | package com.fasterxml.jackson.databind.objectid;
import java.util.*;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.core.*;
import com.fasterxml.jackson.databind.*;
public class Objecid1083Test extends BaseMapTest
{
public static class JsonRoot {
public final List<JsonSchema> schemas = new ArrayList<JsonSchema>();
}
@JsonTypeInfo(
use = JsonTypeInfo.Id.NAME,
property = "type",
defaultImpl = JsonMapSchema.class)
@JsonSubTypes({
@JsonSubTypes.Type(value = JsonMapSchema.class, name = "map"),
@JsonSubTypes.Type(value = JsonJdbcSchema.class, name = "jdbc") })
public static abstract class JsonSchema {
public String name;
}
static class JsonMapSchema extends JsonSchema { }
static class JsonJdbcSchema extends JsonSchema { }
/*
/*****************************************************
/* Unit tests, external id deserialization
/*****************************************************
*/
public void testSimple() throws Exception {
final ObjectMapper mapper = new ObjectMapper();
final String json = aposToQuotes("{'schemas': [{\n"
+ " 'name': 'FoodMart'\n"
+ "}]}\n");
mapper.readValue(json, JsonRoot.class);
}
} |
|
@Test
public void testMinimizeMaximize()
throws FunctionEvaluationException, ConvergenceException {
// the following function has 4 local extrema:
final double xM = -3.841947088256863675365;
final double yM = -1.391745200270734924416;
final double xP = 0.2286682237349059125691;
final double yP = -yM;
final double valueXmYm = 0.2373295333134216789769; // local maximum
final double valueXmYp = -valueXmYm; // local minimum
final double valueXpYm = -0.7290400707055187115322; // global minimum
final double valueXpYp = -valueXpYm; // global maximum
MultivariateRealFunction fourExtrema = new MultivariateRealFunction() {
private static final long serialVersionUID = -7039124064449091152L;
public double value(double[] variables) throws FunctionEvaluationException {
final double x = variables[0];
final double y = variables[1];
return ((x == 0) || (y == 0)) ? 0 : (Math.atan(x) * Math.atan(x + 2) * Math.atan(y) * Math.atan(y) / (x * y));
}
};
MultiDirectional optimizer = new MultiDirectional();
optimizer.setConvergenceChecker(new SimpleScalarValueChecker(1.0e-11, 1.0e-30));
optimizer.setMaxIterations(200);
optimizer.setStartConfiguration(new double[] { 0.2, 0.2 });
RealPointValuePair optimum;
// minimization
optimum = optimizer.optimize(fourExtrema, GoalType.MINIMIZE, new double[] { -3.0, 0 });
Assert.assertEquals(xM, optimum.getPoint()[0], 4.0e-6);
Assert.assertEquals(yP, optimum.getPoint()[1], 3.0e-6);
Assert.assertEquals(valueXmYp, optimum.getValue(), 8.0e-13);
Assert.assertTrue(optimizer.getEvaluations() > 120);
Assert.assertTrue(optimizer.getEvaluations() < 150);
optimum = optimizer.optimize(fourExtrema, GoalType.MINIMIZE, new double[] { +1, 0 });
Assert.assertEquals(xP, optimum.getPoint()[0], 2.0e-8);
Assert.assertEquals(yM, optimum.getPoint()[1], 3.0e-6);
Assert.assertEquals(valueXpYm, optimum.getValue(), 2.0e-12);
Assert.assertTrue(optimizer.getEvaluations() > 120);
Assert.assertTrue(optimizer.getEvaluations() < 150);
// maximization
optimum = optimizer.optimize(fourExtrema, GoalType.MAXIMIZE, new double[] { -3.0, 0.0 });
Assert.assertEquals(xM, optimum.getPoint()[0], 7.0e-7);
Assert.assertEquals(yM, optimum.getPoint()[1], 3.0e-7);
Assert.assertEquals(valueXmYm, optimum.getValue(), 2.0e-14);
Assert.assertTrue(optimizer.getEvaluations() > 120);
Assert.assertTrue(optimizer.getEvaluations() < 150);
optimizer.setConvergenceChecker(new SimpleScalarValueChecker(1.0e-15, 1.0e-30));
optimum = optimizer.optimize(fourExtrema, GoalType.MAXIMIZE, new double[] { +1, 0 });
Assert.assertEquals(xP, optimum.getPoint()[0], 2.0e-8);
Assert.assertEquals(yP, optimum.getPoint()[1], 3.0e-6);
Assert.assertEquals(valueXpYp, optimum.getValue(), 2.0e-12);
Assert.assertTrue(optimizer.getEvaluations() > 180);
Assert.assertTrue(optimizer.getEvaluations() < 220);
} | org.apache.commons.math.optimization.direct.MultiDirectionalTest::testMinimizeMaximize | src/test/java/org/apache/commons/math/optimization/direct/MultiDirectionalTest.java | 127 | src/test/java/org/apache/commons/math/optimization/direct/MultiDirectionalTest.java | testMinimizeMaximize | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.math.optimization.direct;
import org.apache.commons.math.ConvergenceException;
import org.apache.commons.math.FunctionEvaluationException;
import org.apache.commons.math.analysis.MultivariateRealFunction;
import org.apache.commons.math.optimization.GoalType;
import org.apache.commons.math.optimization.OptimizationException;
import org.apache.commons.math.optimization.RealPointValuePair;
import org.apache.commons.math.optimization.SimpleScalarValueChecker;
import org.junit.Assert;
import org.junit.Test;
public class MultiDirectionalTest {
@Test
public void testFunctionEvaluationExceptions() {
MultivariateRealFunction wrong =
new MultivariateRealFunction() {
private static final long serialVersionUID = 4751314470965489371L;
public double value(double[] x) throws FunctionEvaluationException {
if (x[0] < 0) {
throw new FunctionEvaluationException(x, "{0}", "oops");
} else if (x[0] > 1) {
throw new FunctionEvaluationException(new RuntimeException("oops"), x);
} else {
return x[0] * (1 - x[0]);
}
}
};
try {
MultiDirectional optimizer = new MultiDirectional(0.9, 1.9);
optimizer.optimize(wrong, GoalType.MINIMIZE, new double[] { -1.0 });
Assert.fail("an exception should have been thrown");
} catch (FunctionEvaluationException ce) {
// expected behavior
Assert.assertNull(ce.getCause());
} catch (Exception e) {
Assert.fail("wrong exception caught: " + e.getMessage());
}
try {
MultiDirectional optimizer = new MultiDirectional(0.9, 1.9);
optimizer.optimize(wrong, GoalType.MINIMIZE, new double[] { +2.0 });
Assert.fail("an exception should have been thrown");
} catch (FunctionEvaluationException ce) {
// expected behavior
Assert.assertNotNull(ce.getCause());
} catch (Exception e) {
Assert.fail("wrong exception caught: " + e.getMessage());
}
}
@Test
public void testMinimizeMaximize()
throws FunctionEvaluationException, ConvergenceException {
// the following function has 4 local extrema:
final double xM = -3.841947088256863675365;
final double yM = -1.391745200270734924416;
final double xP = 0.2286682237349059125691;
final double yP = -yM;
final double valueXmYm = 0.2373295333134216789769; // local maximum
final double valueXmYp = -valueXmYm; // local minimum
final double valueXpYm = -0.7290400707055187115322; // global minimum
final double valueXpYp = -valueXpYm; // global maximum
MultivariateRealFunction fourExtrema = new MultivariateRealFunction() {
private static final long serialVersionUID = -7039124064449091152L;
public double value(double[] variables) throws FunctionEvaluationException {
final double x = variables[0];
final double y = variables[1];
return ((x == 0) || (y == 0)) ? 0 : (Math.atan(x) * Math.atan(x + 2) * Math.atan(y) * Math.atan(y) / (x * y));
}
};
MultiDirectional optimizer = new MultiDirectional();
optimizer.setConvergenceChecker(new SimpleScalarValueChecker(1.0e-11, 1.0e-30));
optimizer.setMaxIterations(200);
optimizer.setStartConfiguration(new double[] { 0.2, 0.2 });
RealPointValuePair optimum;
// minimization
optimum = optimizer.optimize(fourExtrema, GoalType.MINIMIZE, new double[] { -3.0, 0 });
Assert.assertEquals(xM, optimum.getPoint()[0], 4.0e-6);
Assert.assertEquals(yP, optimum.getPoint()[1], 3.0e-6);
Assert.assertEquals(valueXmYp, optimum.getValue(), 8.0e-13);
Assert.assertTrue(optimizer.getEvaluations() > 120);
Assert.assertTrue(optimizer.getEvaluations() < 150);
optimum = optimizer.optimize(fourExtrema, GoalType.MINIMIZE, new double[] { +1, 0 });
Assert.assertEquals(xP, optimum.getPoint()[0], 2.0e-8);
Assert.assertEquals(yM, optimum.getPoint()[1], 3.0e-6);
Assert.assertEquals(valueXpYm, optimum.getValue(), 2.0e-12);
Assert.assertTrue(optimizer.getEvaluations() > 120);
Assert.assertTrue(optimizer.getEvaluations() < 150);
// maximization
optimum = optimizer.optimize(fourExtrema, GoalType.MAXIMIZE, new double[] { -3.0, 0.0 });
Assert.assertEquals(xM, optimum.getPoint()[0], 7.0e-7);
Assert.assertEquals(yM, optimum.getPoint()[1], 3.0e-7);
Assert.assertEquals(valueXmYm, optimum.getValue(), 2.0e-14);
Assert.assertTrue(optimizer.getEvaluations() > 120);
Assert.assertTrue(optimizer.getEvaluations() < 150);
optimizer.setConvergenceChecker(new SimpleScalarValueChecker(1.0e-15, 1.0e-30));
optimum = optimizer.optimize(fourExtrema, GoalType.MAXIMIZE, new double[] { +1, 0 });
Assert.assertEquals(xP, optimum.getPoint()[0], 2.0e-8);
Assert.assertEquals(yP, optimum.getPoint()[1], 3.0e-6);
Assert.assertEquals(valueXpYp, optimum.getValue(), 2.0e-12);
Assert.assertTrue(optimizer.getEvaluations() > 180);
Assert.assertTrue(optimizer.getEvaluations() < 220);
}
@Test
public void testRosenbrock()
throws FunctionEvaluationException, ConvergenceException {
MultivariateRealFunction rosenbrock =
new MultivariateRealFunction() {
private static final long serialVersionUID = -9044950469615237490L;
public double value(double[] x) throws FunctionEvaluationException {
++count;
double a = x[1] - x[0] * x[0];
double b = 1.0 - x[0];
return 100 * a * a + b * b;
}
};
count = 0;
MultiDirectional optimizer = new MultiDirectional();
optimizer.setConvergenceChecker(new SimpleScalarValueChecker(-1, 1.0e-3));
optimizer.setMaxIterations(100);
optimizer.setStartConfiguration(new double[][] {
{ -1.2, 1.0 }, { 0.9, 1.2 } , { 3.5, -2.3 }
});
RealPointValuePair optimum =
optimizer.optimize(rosenbrock, GoalType.MINIMIZE, new double[] { -1.2, 1.0 });
Assert.assertEquals(count, optimizer.getEvaluations());
Assert.assertTrue(optimizer.getEvaluations() > 50);
Assert.assertTrue(optimizer.getEvaluations() < 100);
Assert.assertTrue(optimum.getValue() > 1.0e-2);
}
@Test
public void testPowell()
throws FunctionEvaluationException, ConvergenceException {
MultivariateRealFunction powell =
new MultivariateRealFunction() {
private static final long serialVersionUID = -832162886102041840L;
public double value(double[] x) throws FunctionEvaluationException {
++count;
double a = x[0] + 10 * x[1];
double b = x[2] - x[3];
double c = x[1] - 2 * x[2];
double d = x[0] - x[3];
return a * a + 5 * b * b + c * c * c * c + 10 * d * d * d * d;
}
};
count = 0;
MultiDirectional optimizer = new MultiDirectional();
optimizer.setConvergenceChecker(new SimpleScalarValueChecker(-1.0, 1.0e-3));
optimizer.setMaxIterations(1000);
RealPointValuePair optimum =
optimizer.optimize(powell, GoalType.MINIMIZE, new double[] { 3.0, -1.0, 0.0, 1.0 });
Assert.assertEquals(count, optimizer.getEvaluations());
Assert.assertTrue(optimizer.getEvaluations() > 800);
Assert.assertTrue(optimizer.getEvaluations() < 900);
Assert.assertTrue(optimum.getValue() > 1.0e-2);
}
@Test
public void testMath283()
throws FunctionEvaluationException, OptimizationException {
// fails because MultiDirectional.iterateSimplex is looping forever
// the while(true) should be replaced with a convergence check
MultiDirectional multiDirectional = new MultiDirectional();
multiDirectional.setMaxIterations(100);
multiDirectional.setMaxEvaluations(1000);
final Gaussian2D function = new Gaussian2D(0.0, 0.0, 1.0);
RealPointValuePair estimate = multiDirectional.optimize(function,
GoalType.MAXIMIZE, function.getMaximumPosition());
final double EPSILON = 1e-5;
final double expectedMaximum = function.getMaximum();
final double actualMaximum = estimate.getValue();
Assert.assertEquals(expectedMaximum, actualMaximum, EPSILON);
final double[] expectedPosition = function.getMaximumPosition();
final double[] actualPosition = estimate.getPoint();
Assert.assertEquals(expectedPosition[0], actualPosition[0], EPSILON );
Assert.assertEquals(expectedPosition[1], actualPosition[1], EPSILON );
}
private static class Gaussian2D implements MultivariateRealFunction {
private final double[] maximumPosition;
private final double std;
public Gaussian2D(double xOpt, double yOpt, double std) {
maximumPosition = new double[] { xOpt, yOpt };
this.std = std;
}
public double getMaximum() {
return value(maximumPosition);
}
public double[] getMaximumPosition() {
return maximumPosition.clone();
}
public double value(double[] point) {
final double x = point[0], y = point[1];
final double twoS2 = 2.0 * std * std;
return 1.0 / (twoS2 * Math.PI) * Math.exp(-(x * x + y * y) / twoS2);
}
}
private int count;
} | // You are a professional Java test case writer, please create a test case named `testMinimizeMaximize` for the issue `Math-MATH-283`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Math-MATH-283
//
// ## Issue-Title:
// MultiDirectional optimzation loops forver if started at the correct solution
//
// ## Issue-Description:
//
// MultiDirectional.iterateSimplex loops forever if the starting point is the correct solution.
//
//
// see the attached test case (testMultiDirectionalCorrectStart) as an example.
//
//
//
//
//
@Test
public void testMinimizeMaximize()
throws FunctionEvaluationException, ConvergenceException {
| 127 | 84 | 68 | src/test/java/org/apache/commons/math/optimization/direct/MultiDirectionalTest.java | src/test/java | ```markdown
## Issue-ID: Math-MATH-283
## Issue-Title:
MultiDirectional optimzation loops forver if started at the correct solution
## Issue-Description:
MultiDirectional.iterateSimplex loops forever if the starting point is the correct solution.
see the attached test case (testMultiDirectionalCorrectStart) as an example.
```
You are a professional Java test case writer, please create a test case named `testMinimizeMaximize` for the issue `Math-MATH-283`, utilizing the provided issue report information and the following function signature.
```java
@Test
public void testMinimizeMaximize()
throws FunctionEvaluationException, ConvergenceException {
```
| 68 | [
"org.apache.commons.math.optimization.direct.MultiDirectional"
] | 7bceba76534ac7dc7561e726cde4d805e5694c2e374f85f211eafd337242ed31 | @Test
public void testMinimizeMaximize()
throws FunctionEvaluationException, ConvergenceException | // You are a professional Java test case writer, please create a test case named `testMinimizeMaximize` for the issue `Math-MATH-283`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Math-MATH-283
//
// ## Issue-Title:
// MultiDirectional optimzation loops forver if started at the correct solution
//
// ## Issue-Description:
//
// MultiDirectional.iterateSimplex loops forever if the starting point is the correct solution.
//
//
// see the attached test case (testMultiDirectionalCorrectStart) as an example.
//
//
//
//
//
| Math | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.math.optimization.direct;
import org.apache.commons.math.ConvergenceException;
import org.apache.commons.math.FunctionEvaluationException;
import org.apache.commons.math.analysis.MultivariateRealFunction;
import org.apache.commons.math.optimization.GoalType;
import org.apache.commons.math.optimization.OptimizationException;
import org.apache.commons.math.optimization.RealPointValuePair;
import org.apache.commons.math.optimization.SimpleScalarValueChecker;
import org.junit.Assert;
import org.junit.Test;
public class MultiDirectionalTest {
@Test
public void testFunctionEvaluationExceptions() {
MultivariateRealFunction wrong =
new MultivariateRealFunction() {
private static final long serialVersionUID = 4751314470965489371L;
public double value(double[] x) throws FunctionEvaluationException {
if (x[0] < 0) {
throw new FunctionEvaluationException(x, "{0}", "oops");
} else if (x[0] > 1) {
throw new FunctionEvaluationException(new RuntimeException("oops"), x);
} else {
return x[0] * (1 - x[0]);
}
}
};
try {
MultiDirectional optimizer = new MultiDirectional(0.9, 1.9);
optimizer.optimize(wrong, GoalType.MINIMIZE, new double[] { -1.0 });
Assert.fail("an exception should have been thrown");
} catch (FunctionEvaluationException ce) {
// expected behavior
Assert.assertNull(ce.getCause());
} catch (Exception e) {
Assert.fail("wrong exception caught: " + e.getMessage());
}
try {
MultiDirectional optimizer = new MultiDirectional(0.9, 1.9);
optimizer.optimize(wrong, GoalType.MINIMIZE, new double[] { +2.0 });
Assert.fail("an exception should have been thrown");
} catch (FunctionEvaluationException ce) {
// expected behavior
Assert.assertNotNull(ce.getCause());
} catch (Exception e) {
Assert.fail("wrong exception caught: " + e.getMessage());
}
}
@Test
public void testMinimizeMaximize()
throws FunctionEvaluationException, ConvergenceException {
// the following function has 4 local extrema:
final double xM = -3.841947088256863675365;
final double yM = -1.391745200270734924416;
final double xP = 0.2286682237349059125691;
final double yP = -yM;
final double valueXmYm = 0.2373295333134216789769; // local maximum
final double valueXmYp = -valueXmYm; // local minimum
final double valueXpYm = -0.7290400707055187115322; // global minimum
final double valueXpYp = -valueXpYm; // global maximum
MultivariateRealFunction fourExtrema = new MultivariateRealFunction() {
private static final long serialVersionUID = -7039124064449091152L;
public double value(double[] variables) throws FunctionEvaluationException {
final double x = variables[0];
final double y = variables[1];
return ((x == 0) || (y == 0)) ? 0 : (Math.atan(x) * Math.atan(x + 2) * Math.atan(y) * Math.atan(y) / (x * y));
}
};
MultiDirectional optimizer = new MultiDirectional();
optimizer.setConvergenceChecker(new SimpleScalarValueChecker(1.0e-11, 1.0e-30));
optimizer.setMaxIterations(200);
optimizer.setStartConfiguration(new double[] { 0.2, 0.2 });
RealPointValuePair optimum;
// minimization
optimum = optimizer.optimize(fourExtrema, GoalType.MINIMIZE, new double[] { -3.0, 0 });
Assert.assertEquals(xM, optimum.getPoint()[0], 4.0e-6);
Assert.assertEquals(yP, optimum.getPoint()[1], 3.0e-6);
Assert.assertEquals(valueXmYp, optimum.getValue(), 8.0e-13);
Assert.assertTrue(optimizer.getEvaluations() > 120);
Assert.assertTrue(optimizer.getEvaluations() < 150);
optimum = optimizer.optimize(fourExtrema, GoalType.MINIMIZE, new double[] { +1, 0 });
Assert.assertEquals(xP, optimum.getPoint()[0], 2.0e-8);
Assert.assertEquals(yM, optimum.getPoint()[1], 3.0e-6);
Assert.assertEquals(valueXpYm, optimum.getValue(), 2.0e-12);
Assert.assertTrue(optimizer.getEvaluations() > 120);
Assert.assertTrue(optimizer.getEvaluations() < 150);
// maximization
optimum = optimizer.optimize(fourExtrema, GoalType.MAXIMIZE, new double[] { -3.0, 0.0 });
Assert.assertEquals(xM, optimum.getPoint()[0], 7.0e-7);
Assert.assertEquals(yM, optimum.getPoint()[1], 3.0e-7);
Assert.assertEquals(valueXmYm, optimum.getValue(), 2.0e-14);
Assert.assertTrue(optimizer.getEvaluations() > 120);
Assert.assertTrue(optimizer.getEvaluations() < 150);
optimizer.setConvergenceChecker(new SimpleScalarValueChecker(1.0e-15, 1.0e-30));
optimum = optimizer.optimize(fourExtrema, GoalType.MAXIMIZE, new double[] { +1, 0 });
Assert.assertEquals(xP, optimum.getPoint()[0], 2.0e-8);
Assert.assertEquals(yP, optimum.getPoint()[1], 3.0e-6);
Assert.assertEquals(valueXpYp, optimum.getValue(), 2.0e-12);
Assert.assertTrue(optimizer.getEvaluations() > 180);
Assert.assertTrue(optimizer.getEvaluations() < 220);
}
@Test
public void testRosenbrock()
throws FunctionEvaluationException, ConvergenceException {
MultivariateRealFunction rosenbrock =
new MultivariateRealFunction() {
private static final long serialVersionUID = -9044950469615237490L;
public double value(double[] x) throws FunctionEvaluationException {
++count;
double a = x[1] - x[0] * x[0];
double b = 1.0 - x[0];
return 100 * a * a + b * b;
}
};
count = 0;
MultiDirectional optimizer = new MultiDirectional();
optimizer.setConvergenceChecker(new SimpleScalarValueChecker(-1, 1.0e-3));
optimizer.setMaxIterations(100);
optimizer.setStartConfiguration(new double[][] {
{ -1.2, 1.0 }, { 0.9, 1.2 } , { 3.5, -2.3 }
});
RealPointValuePair optimum =
optimizer.optimize(rosenbrock, GoalType.MINIMIZE, new double[] { -1.2, 1.0 });
Assert.assertEquals(count, optimizer.getEvaluations());
Assert.assertTrue(optimizer.getEvaluations() > 50);
Assert.assertTrue(optimizer.getEvaluations() < 100);
Assert.assertTrue(optimum.getValue() > 1.0e-2);
}
@Test
public void testPowell()
throws FunctionEvaluationException, ConvergenceException {
MultivariateRealFunction powell =
new MultivariateRealFunction() {
private static final long serialVersionUID = -832162886102041840L;
public double value(double[] x) throws FunctionEvaluationException {
++count;
double a = x[0] + 10 * x[1];
double b = x[2] - x[3];
double c = x[1] - 2 * x[2];
double d = x[0] - x[3];
return a * a + 5 * b * b + c * c * c * c + 10 * d * d * d * d;
}
};
count = 0;
MultiDirectional optimizer = new MultiDirectional();
optimizer.setConvergenceChecker(new SimpleScalarValueChecker(-1.0, 1.0e-3));
optimizer.setMaxIterations(1000);
RealPointValuePair optimum =
optimizer.optimize(powell, GoalType.MINIMIZE, new double[] { 3.0, -1.0, 0.0, 1.0 });
Assert.assertEquals(count, optimizer.getEvaluations());
Assert.assertTrue(optimizer.getEvaluations() > 800);
Assert.assertTrue(optimizer.getEvaluations() < 900);
Assert.assertTrue(optimum.getValue() > 1.0e-2);
}
@Test
public void testMath283()
throws FunctionEvaluationException, OptimizationException {
// fails because MultiDirectional.iterateSimplex is looping forever
// the while(true) should be replaced with a convergence check
MultiDirectional multiDirectional = new MultiDirectional();
multiDirectional.setMaxIterations(100);
multiDirectional.setMaxEvaluations(1000);
final Gaussian2D function = new Gaussian2D(0.0, 0.0, 1.0);
RealPointValuePair estimate = multiDirectional.optimize(function,
GoalType.MAXIMIZE, function.getMaximumPosition());
final double EPSILON = 1e-5;
final double expectedMaximum = function.getMaximum();
final double actualMaximum = estimate.getValue();
Assert.assertEquals(expectedMaximum, actualMaximum, EPSILON);
final double[] expectedPosition = function.getMaximumPosition();
final double[] actualPosition = estimate.getPoint();
Assert.assertEquals(expectedPosition[0], actualPosition[0], EPSILON );
Assert.assertEquals(expectedPosition[1], actualPosition[1], EPSILON );
}
private static class Gaussian2D implements MultivariateRealFunction {
private final double[] maximumPosition;
private final double std;
public Gaussian2D(double xOpt, double yOpt, double std) {
maximumPosition = new double[] { xOpt, yOpt };
this.std = std;
}
public double getMaximum() {
return value(maximumPosition);
}
public double[] getMaximumPosition() {
return maximumPosition.clone();
}
public double value(double[] point) {
final double x = point[0], y = point[1];
final double twoS2 = 2.0 * std * std;
return 1.0 / (twoS2 * Math.PI) * Math.exp(-(x * x + y * y) / twoS2);
}
}
private int count;
} |
||
public void testContainsAnyCharArrayWithSupplementaryChars() {
assertEquals(true, StringUtils.containsAny(CharU20000 + CharU20001, CharU20000.toCharArray()));
assertEquals(true, StringUtils.containsAny(CharU20000 + CharU20001, CharU20001.toCharArray()));
assertEquals(true, StringUtils.containsAny(CharU20000, CharU20000.toCharArray()));
// Sanity check:
assertEquals(-1, CharU20000.indexOf(CharU20001));
assertEquals(0, CharU20000.indexOf(CharU20001.charAt(0)));
assertEquals(-1, CharU20000.indexOf(CharU20001.charAt(1)));
// Test:
assertEquals(false, StringUtils.containsAny(CharU20000, CharU20001.toCharArray()));
assertEquals(false, StringUtils.containsAny(CharU20001, CharU20000.toCharArray()));
} | org.apache.commons.lang3.StringUtilsEqualsIndexOfTest::testContainsAnyCharArrayWithSupplementaryChars | src/test/java/org/apache/commons/lang3/StringUtilsEqualsIndexOfTest.java | 697 | src/test/java/org/apache/commons/lang3/StringUtilsEqualsIndexOfTest.java | testContainsAnyCharArrayWithSupplementaryChars | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.lang3;
import java.util.Locale;
import junit.framework.TestCase;
/**
* Unit tests {@link org.apache.commons.lang3.StringUtils} - Substring methods
*
* @author Apache Software Foundation
* @author <a href="mailto:[email protected]">Ringo De Smet</a>
* @author Phil Steitz
* @version $Id$
*/
public class StringUtilsEqualsIndexOfTest extends TestCase {
private static final String FOO = "foo";
private static final String BAR = "bar";
private static final String FOOBAR = "foobar";
private static final String[] FOOBAR_SUB_ARRAY = new String[] {"ob", "ba"};
/**
* Supplementary character U+20000
* See http://java.sun.com/developer/technicalArticles/Intl/Supplementary/
*/
private static final String CharU20000 = "\uD840\uDC00";
/**
* Supplementary character U+20001
* See http://java.sun.com/developer/technicalArticles/Intl/Supplementary/
*/
private static final String CharU20001 = "\uD840\uDC01";
public StringUtilsEqualsIndexOfTest(String name) {
super(name);
}
//-----------------------------------------------------------------------
public void testEquals() {
assertEquals(true, StringUtils.equals(null, null));
assertEquals(true, StringUtils.equals(FOO, FOO));
assertEquals(true, StringUtils.equals(FOO, new String(new char[] { 'f', 'o', 'o' })));
assertEquals(false, StringUtils.equals(FOO, new String(new char[] { 'f', 'O', 'O' })));
assertEquals(false, StringUtils.equals(FOO, BAR));
assertEquals(false, StringUtils.equals(FOO, null));
assertEquals(false, StringUtils.equals(null, FOO));
}
public void testEqualsIgnoreCase() {
assertEquals(true, StringUtils.equalsIgnoreCase(null, null));
assertEquals(true, StringUtils.equalsIgnoreCase(FOO, FOO));
assertEquals(true, StringUtils.equalsIgnoreCase(FOO, new String(new char[] { 'f', 'o', 'o' })));
assertEquals(true, StringUtils.equalsIgnoreCase(FOO, new String(new char[] { 'f', 'O', 'O' })));
assertEquals(false, StringUtils.equalsIgnoreCase(FOO, BAR));
assertEquals(false, StringUtils.equalsIgnoreCase(FOO, null));
assertEquals(false, StringUtils.equalsIgnoreCase(null, FOO));
}
//-----------------------------------------------------------------------
public void testIndexOf_char() {
assertEquals(-1, StringUtils.indexOf(null, ' '));
assertEquals(-1, StringUtils.indexOf("", ' '));
assertEquals(0, StringUtils.indexOf("aabaabaa", 'a'));
assertEquals(2, StringUtils.indexOf("aabaabaa", 'b'));
}
public void testIndexOf_charInt() {
assertEquals(-1, StringUtils.indexOf(null, ' ', 0));
assertEquals(-1, StringUtils.indexOf(null, ' ', -1));
assertEquals(-1, StringUtils.indexOf("", ' ', 0));
assertEquals(-1, StringUtils.indexOf("", ' ', -1));
assertEquals(0, StringUtils.indexOf("aabaabaa", 'a', 0));
assertEquals(2, StringUtils.indexOf("aabaabaa", 'b', 0));
assertEquals(5, StringUtils.indexOf("aabaabaa", 'b', 3));
assertEquals(-1, StringUtils.indexOf("aabaabaa", 'b', 9));
assertEquals(2, StringUtils.indexOf("aabaabaa", 'b', -1));
}
public void testIndexOf_String() {
assertEquals(-1, StringUtils.indexOf(null, null));
assertEquals(-1, StringUtils.indexOf("", null));
assertEquals(0, StringUtils.indexOf("", ""));
assertEquals(0, StringUtils.indexOf("aabaabaa", "a"));
assertEquals(2, StringUtils.indexOf("aabaabaa", "b"));
assertEquals(1, StringUtils.indexOf("aabaabaa", "ab"));
assertEquals(0, StringUtils.indexOf("aabaabaa", ""));
}
public void testIndexOfIgnoreCase_String() {
assertEquals(-1, StringUtils.indexOfIgnoreCase(null, null));
assertEquals(-1, StringUtils.indexOfIgnoreCase(null, ""));
assertEquals(-1, StringUtils.indexOfIgnoreCase("", null));
assertEquals(0, StringUtils.indexOfIgnoreCase("", ""));
assertEquals(0, StringUtils.indexOfIgnoreCase("aabaabaa", "a"));
assertEquals(0, StringUtils.indexOfIgnoreCase("aabaabaa", "A"));
assertEquals(2, StringUtils.indexOfIgnoreCase("aabaabaa", "b"));
assertEquals(2, StringUtils.indexOfIgnoreCase("aabaabaa", "B"));
assertEquals(1, StringUtils.indexOfIgnoreCase("aabaabaa", "ab"));
assertEquals(1, StringUtils.indexOfIgnoreCase("aabaabaa", "AB"));
assertEquals(0, StringUtils.indexOfIgnoreCase("aabaabaa", ""));
}
public void testIndexOfIgnoreCase_StringInt() {
assertEquals(1, StringUtils.indexOfIgnoreCase("aabaabaa", "AB", -1));
assertEquals(1, StringUtils.indexOfIgnoreCase("aabaabaa", "AB", 0));
assertEquals(1, StringUtils.indexOfIgnoreCase("aabaabaa", "AB", 1));
assertEquals(4, StringUtils.indexOfIgnoreCase("aabaabaa", "AB", 2));
assertEquals(4, StringUtils.indexOfIgnoreCase("aabaabaa", "AB", 3));
assertEquals(4, StringUtils.indexOfIgnoreCase("aabaabaa", "AB", 4));
assertEquals(-1, StringUtils.indexOfIgnoreCase("aabaabaa", "AB", 5));
assertEquals(-1, StringUtils.indexOfIgnoreCase("aabaabaa", "AB", 6));
assertEquals(-1, StringUtils.indexOfIgnoreCase("aabaabaa", "AB", 7));
assertEquals(-1, StringUtils.indexOfIgnoreCase("aabaabaa", "AB", 8));
assertEquals(1, StringUtils.indexOfIgnoreCase("aab", "AB", 1));
assertEquals(5, StringUtils.indexOfIgnoreCase("aabaabaa", "", 5));
assertEquals(-1, StringUtils.indexOfIgnoreCase("ab", "AAB", 0));
assertEquals(-1, StringUtils.indexOfIgnoreCase("aab", "AAB", 1));
}
public void testOrdinalIndexOf() {
assertEquals(-1, StringUtils.ordinalIndexOf(null, null, Integer.MIN_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("", null, Integer.MIN_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("", "", Integer.MIN_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "a", Integer.MIN_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "b", Integer.MIN_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "ab", Integer.MIN_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "", Integer.MIN_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf(null, null, -1));
assertEquals(-1, StringUtils.ordinalIndexOf("", null, -1));
assertEquals(-1, StringUtils.ordinalIndexOf("", "", -1));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "a", -1));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "b", -1));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "ab", -1));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "", -1));
assertEquals(-1, StringUtils.ordinalIndexOf(null, null, 0));
assertEquals(-1, StringUtils.ordinalIndexOf("", null, 0));
assertEquals(-1, StringUtils.ordinalIndexOf("", "", 0));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "a", 0));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "b", 0));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "ab", 0));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "", 0));
assertEquals(-1, StringUtils.ordinalIndexOf(null, null, 1));
assertEquals(-1, StringUtils.ordinalIndexOf("", null, 1));
assertEquals(0, StringUtils.ordinalIndexOf("", "", 1));
assertEquals(0, StringUtils.ordinalIndexOf("aabaabaa", "a", 1));
assertEquals(2, StringUtils.ordinalIndexOf("aabaabaa", "b", 1));
assertEquals(1, StringUtils.ordinalIndexOf("aabaabaa", "ab", 1));
assertEquals(0, StringUtils.ordinalIndexOf("aabaabaa", "", 1));
assertEquals(-1, StringUtils.ordinalIndexOf(null, null, 2));
assertEquals(-1, StringUtils.ordinalIndexOf("", null, 2));
assertEquals(0, StringUtils.ordinalIndexOf("", "", 2));
assertEquals(1, StringUtils.ordinalIndexOf("aabaabaa", "a", 2));
assertEquals(5, StringUtils.ordinalIndexOf("aabaabaa", "b", 2));
assertEquals(4, StringUtils.ordinalIndexOf("aabaabaa", "ab", 2));
assertEquals(0, StringUtils.ordinalIndexOf("aabaabaa", "", 2));
assertEquals(-1, StringUtils.ordinalIndexOf(null, null, Integer.MAX_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("", null, Integer.MAX_VALUE));
assertEquals(0, StringUtils.ordinalIndexOf("", "", Integer.MAX_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "a", Integer.MAX_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "b", Integer.MAX_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "ab", Integer.MAX_VALUE));
assertEquals(0, StringUtils.ordinalIndexOf("aabaabaa", "", Integer.MAX_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 0));
assertEquals(0, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 1));
assertEquals(1, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 2));
assertEquals(2, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 3));
assertEquals(3, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 4));
assertEquals(4, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 5));
assertEquals(5, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 6));
assertEquals(6, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 7));
assertEquals(7, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 8));
assertEquals(8, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 9));
assertEquals(-1, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 10));
}
public void testIndexOf_StringInt() {
assertEquals(-1, StringUtils.indexOf(null, null, 0));
assertEquals(-1, StringUtils.indexOf(null, null, -1));
assertEquals(-1, StringUtils.indexOf(null, "", 0));
assertEquals(-1, StringUtils.indexOf(null, "", -1));
assertEquals(-1, StringUtils.indexOf("", null, 0));
assertEquals(-1, StringUtils.indexOf("", null, -1));
assertEquals(0, StringUtils.indexOf("", "", 0));
assertEquals(0, StringUtils.indexOf("", "", -1));
assertEquals(0, StringUtils.indexOf("", "", 9));
assertEquals(0, StringUtils.indexOf("abc", "", 0));
assertEquals(0, StringUtils.indexOf("abc", "", -1));
assertEquals(3, StringUtils.indexOf("abc", "", 9));
assertEquals(3, StringUtils.indexOf("abc", "", 3));
assertEquals(0, StringUtils.indexOf("aabaabaa", "a", 0));
assertEquals(2, StringUtils.indexOf("aabaabaa", "b", 0));
assertEquals(1, StringUtils.indexOf("aabaabaa", "ab", 0));
assertEquals(5, StringUtils.indexOf("aabaabaa", "b", 3));
assertEquals(-1, StringUtils.indexOf("aabaabaa", "b", 9));
assertEquals(2, StringUtils.indexOf("aabaabaa", "b", -1));
assertEquals(2,StringUtils.indexOf("aabaabaa", "", 2));
}
//-----------------------------------------------------------------------
public void testLastIndexOf_char() {
assertEquals(-1, StringUtils.lastIndexOf(null, ' '));
assertEquals(-1, StringUtils.lastIndexOf("", ' '));
assertEquals(7, StringUtils.lastIndexOf("aabaabaa", 'a'));
assertEquals(5, StringUtils.lastIndexOf("aabaabaa", 'b'));
}
public void testLastIndexOf_charInt() {
assertEquals(-1, StringUtils.lastIndexOf(null, ' ', 0));
assertEquals(-1, StringUtils.lastIndexOf(null, ' ', -1));
assertEquals(-1, StringUtils.lastIndexOf("", ' ', 0));
assertEquals(-1, StringUtils.lastIndexOf("", ' ', -1));
assertEquals(7, StringUtils.lastIndexOf("aabaabaa", 'a', 8));
assertEquals(5, StringUtils.lastIndexOf("aabaabaa", 'b', 8));
assertEquals(2, StringUtils.lastIndexOf("aabaabaa", 'b', 3));
assertEquals(5, StringUtils.lastIndexOf("aabaabaa", 'b', 9));
assertEquals(-1, StringUtils.lastIndexOf("aabaabaa", 'b', -1));
assertEquals(0, StringUtils.lastIndexOf("aabaabaa", 'a', 0));
}
public void testLastIndexOf_String() {
assertEquals(-1, StringUtils.lastIndexOf(null, null));
assertEquals(-1, StringUtils.lastIndexOf("", null));
assertEquals(-1, StringUtils.lastIndexOf("", "a"));
assertEquals(0, StringUtils.lastIndexOf("", ""));
assertEquals(8, StringUtils.lastIndexOf("aabaabaa", ""));
assertEquals(7, StringUtils.lastIndexOf("aabaabaa", "a"));
assertEquals(5, StringUtils.lastIndexOf("aabaabaa", "b"));
assertEquals(4, StringUtils.lastIndexOf("aabaabaa", "ab"));
}
public void testLastOrdinalIndexOf() {
assertEquals(-1, StringUtils.lastOrdinalIndexOf(null, "*", 42) );
assertEquals(-1, StringUtils.lastOrdinalIndexOf("*", null, 42) );
assertEquals(0, StringUtils.lastOrdinalIndexOf("", "", 42) );
assertEquals(7, StringUtils.lastOrdinalIndexOf("aabaabaa", "a", 1) );
assertEquals(6, StringUtils.lastOrdinalIndexOf("aabaabaa", "a", 2) );
assertEquals(5, StringUtils.lastOrdinalIndexOf("aabaabaa", "b", 1) );
assertEquals(2, StringUtils.lastOrdinalIndexOf("aabaabaa", "b", 2) );
assertEquals(4, StringUtils.lastOrdinalIndexOf("aabaabaa", "ab", 1) );
assertEquals(1, StringUtils.lastOrdinalIndexOf("aabaabaa", "ab", 2) );
assertEquals(8, StringUtils.lastOrdinalIndexOf("aabaabaa", "", 1) );
assertEquals(8, StringUtils.lastOrdinalIndexOf("aabaabaa", "", 2) );
}
public void testLastIndexOf_StringInt() {
assertEquals(-1, StringUtils.lastIndexOf(null, null, 0));
assertEquals(-1, StringUtils.lastIndexOf(null, null, -1));
assertEquals(-1, StringUtils.lastIndexOf(null, "", 0));
assertEquals(-1, StringUtils.lastIndexOf(null, "", -1));
assertEquals(-1, StringUtils.lastIndexOf("", null, 0));
assertEquals(-1, StringUtils.lastIndexOf("", null, -1));
assertEquals(0, StringUtils.lastIndexOf("", "", 0));
assertEquals(-1, StringUtils.lastIndexOf("", "", -1));
assertEquals(0, StringUtils.lastIndexOf("", "", 9));
assertEquals(0, StringUtils.lastIndexOf("abc", "", 0));
assertEquals(-1, StringUtils.lastIndexOf("abc", "", -1));
assertEquals(3, StringUtils.lastIndexOf("abc", "", 9));
assertEquals(7, StringUtils.lastIndexOf("aabaabaa", "a", 8));
assertEquals(5, StringUtils.lastIndexOf("aabaabaa", "b", 8));
assertEquals(4, StringUtils.lastIndexOf("aabaabaa", "ab", 8));
assertEquals(2, StringUtils.lastIndexOf("aabaabaa", "b", 3));
assertEquals(5, StringUtils.lastIndexOf("aabaabaa", "b", 9));
assertEquals(-1, StringUtils.lastIndexOf("aabaabaa", "b", -1));
assertEquals(-1, StringUtils.lastIndexOf("aabaabaa", "b", 0));
assertEquals(0, StringUtils.lastIndexOf("aabaabaa", "a", 0));
}
public void testLastIndexOfIgnoreCase_String() {
assertEquals(-1, StringUtils.lastIndexOfIgnoreCase(null, null));
assertEquals(-1, StringUtils.lastIndexOfIgnoreCase("", null));
assertEquals(-1, StringUtils.lastIndexOfIgnoreCase(null, ""));
assertEquals(-1, StringUtils.lastIndexOfIgnoreCase("", "a"));
assertEquals(0, StringUtils.lastIndexOfIgnoreCase("", ""));
assertEquals(8, StringUtils.lastIndexOfIgnoreCase("aabaabaa", ""));
assertEquals(7, StringUtils.lastIndexOfIgnoreCase("aabaabaa", "a"));
assertEquals(7, StringUtils.lastIndexOfIgnoreCase("aabaabaa", "A"));
assertEquals(5, StringUtils.lastIndexOfIgnoreCase("aabaabaa", "b"));
assertEquals(5, StringUtils.lastIndexOfIgnoreCase("aabaabaa", "B"));
assertEquals(4, StringUtils.lastIndexOfIgnoreCase("aabaabaa", "ab"));
assertEquals(4, StringUtils.lastIndexOfIgnoreCase("aabaabaa", "AB"));
assertEquals(-1, StringUtils.lastIndexOfIgnoreCase("ab", "AAB"));
assertEquals(0, StringUtils.lastIndexOfIgnoreCase("aab", "AAB"));
}
public void testLastIndexOfIgnoreCase_StringInt() {
assertEquals(-1, StringUtils.lastIndexOfIgnoreCase(null, null, 0));
assertEquals(-1, StringUtils.lastIndexOfIgnoreCase(null, null, -1));
assertEquals(-1, StringUtils.lastIndexOfIgnoreCase(null, "", 0));
assertEquals(-1, StringUtils.lastIndexOfIgnoreCase(null, "", -1));
assertEquals(-1, StringUtils.lastIndexOfIgnoreCase("", null, 0));
assertEquals(-1, StringUtils.lastIndexOfIgnoreCase("", null, -1));
assertEquals(0, StringUtils.lastIndexOfIgnoreCase("", "", 0));
assertEquals(-1, StringUtils.lastIndexOfIgnoreCase("", "", -1));
assertEquals(0, StringUtils.lastIndexOfIgnoreCase("", "", 9));
assertEquals(0, StringUtils.lastIndexOfIgnoreCase("abc", "", 0));
assertEquals(-1, StringUtils.lastIndexOfIgnoreCase("abc", "", -1));
assertEquals(3, StringUtils.lastIndexOfIgnoreCase("abc", "", 9));
assertEquals(7, StringUtils.lastIndexOfIgnoreCase("aabaabaa", "A", 8));
assertEquals(5, StringUtils.lastIndexOfIgnoreCase("aabaabaa", "B", 8));
assertEquals(4, StringUtils.lastIndexOfIgnoreCase("aabaabaa", "AB", 8));
assertEquals(2, StringUtils.lastIndexOfIgnoreCase("aabaabaa", "B", 3));
assertEquals(5, StringUtils.lastIndexOfIgnoreCase("aabaabaa", "B", 9));
assertEquals(-1, StringUtils.lastIndexOfIgnoreCase("aabaabaa", "B", -1));
assertEquals(-1, StringUtils.lastIndexOfIgnoreCase("aabaabaa", "B", 0));
assertEquals(0, StringUtils.lastIndexOfIgnoreCase("aabaabaa", "A", 0));
assertEquals(1, StringUtils.lastIndexOfIgnoreCase("aab", "AB", 1));
}
//-----------------------------------------------------------------------
public void testContainsChar() {
assertEquals(false, StringUtils.contains(null, ' '));
assertEquals(false, StringUtils.contains("", ' '));
assertEquals(false, StringUtils.contains("",null));
assertEquals(false, StringUtils.contains(null,null));
assertEquals(true, StringUtils.contains("abc", 'a'));
assertEquals(true, StringUtils.contains("abc", 'b'));
assertEquals(true, StringUtils.contains("abc", 'c'));
assertEquals(false, StringUtils.contains("abc", 'z'));
}
public void testContainsString() {
assertEquals(false, StringUtils.contains(null, null));
assertEquals(false, StringUtils.contains(null, ""));
assertEquals(false, StringUtils.contains(null, "a"));
assertEquals(false, StringUtils.contains("", null));
assertEquals(true, StringUtils.contains("", ""));
assertEquals(false, StringUtils.contains("", "a"));
assertEquals(true, StringUtils.contains("abc", "a"));
assertEquals(true, StringUtils.contains("abc", "b"));
assertEquals(true, StringUtils.contains("abc", "c"));
assertEquals(true, StringUtils.contains("abc", "abc"));
assertEquals(false, StringUtils.contains("abc", "z"));
}
public void testContainsIgnoreCase_StringString() {
assertFalse(StringUtils.containsIgnoreCase(null, null));
// Null tests
assertFalse(StringUtils.containsIgnoreCase(null, ""));
assertFalse(StringUtils.containsIgnoreCase(null, "a"));
assertFalse(StringUtils.containsIgnoreCase(null, "abc"));
assertFalse(StringUtils.containsIgnoreCase("", null));
assertFalse(StringUtils.containsIgnoreCase("a", null));
assertFalse(StringUtils.containsIgnoreCase("abc", null));
// Match len = 0
assertTrue(StringUtils.containsIgnoreCase("", ""));
assertTrue(StringUtils.containsIgnoreCase("a", ""));
assertTrue(StringUtils.containsIgnoreCase("abc", ""));
// Match len = 1
assertFalse(StringUtils.containsIgnoreCase("", "a"));
assertTrue(StringUtils.containsIgnoreCase("a", "a"));
assertTrue(StringUtils.containsIgnoreCase("abc", "a"));
assertFalse(StringUtils.containsIgnoreCase("", "A"));
assertTrue(StringUtils.containsIgnoreCase("a", "A"));
assertTrue(StringUtils.containsIgnoreCase("abc", "A"));
// Match len > 1
assertFalse(StringUtils.containsIgnoreCase("", "abc"));
assertFalse(StringUtils.containsIgnoreCase("a", "abc"));
assertTrue(StringUtils.containsIgnoreCase("xabcz", "abc"));
assertFalse(StringUtils.containsIgnoreCase("", "ABC"));
assertFalse(StringUtils.containsIgnoreCase("a", "ABC"));
assertTrue(StringUtils.containsIgnoreCase("xabcz", "ABC"));
}
public void testContainsIgnoreCase_LocaleIndependence() {
Locale orig = Locale.getDefault();
Locale[] locales = { Locale.ENGLISH, new Locale("tr"), Locale.getDefault() };
String[][] tdata = {
{ "i", "I" },
{ "I", "i" },
{ "\u03C2", "\u03C3" },
{ "\u03A3", "\u03C2" },
{ "\u03A3", "\u03C3" },
};
String[][] fdata = {
{ "\u00DF", "SS" },
};
try {
for (int i = 0; i < locales.length; i++) {
Locale.setDefault(locales[i]);
for (int j = 0; j < tdata.length; j++) {
assertTrue(Locale.getDefault() + ": " + j + " " + tdata[j][0] + " " + tdata[j][1], StringUtils
.containsIgnoreCase(tdata[j][0], tdata[j][1]));
}
for (int j = 0; j < fdata.length; j++) {
assertFalse(Locale.getDefault() + ": " + j + " " + fdata[j][0] + " " + fdata[j][1], StringUtils
.containsIgnoreCase(fdata[j][0], fdata[j][1]));
}
}
} finally {
Locale.setDefault(orig);
}
}
// -----------------------------------------------------------------------
public void testIndexOfAny_StringStringarray() {
assertEquals(-1, StringUtils.indexOfAny(null, (String[]) null));
assertEquals(-1, StringUtils.indexOfAny(null, FOOBAR_SUB_ARRAY));
assertEquals(-1, StringUtils.indexOfAny(FOOBAR, (String[]) null));
assertEquals(2, StringUtils.indexOfAny(FOOBAR, FOOBAR_SUB_ARRAY));
assertEquals(-1, StringUtils.indexOfAny(FOOBAR, new String[0]));
assertEquals(-1, StringUtils.indexOfAny(null, new String[0]));
assertEquals(-1, StringUtils.indexOfAny("", new String[0]));
assertEquals(-1, StringUtils.indexOfAny(FOOBAR, new String[] {"llll"}));
assertEquals(0, StringUtils.indexOfAny(FOOBAR, new String[] {""}));
assertEquals(0, StringUtils.indexOfAny("", new String[] {""}));
assertEquals(-1, StringUtils.indexOfAny("", new String[] {"a"}));
assertEquals(-1, StringUtils.indexOfAny("", new String[] {null}));
assertEquals(-1, StringUtils.indexOfAny(FOOBAR, new String[] {null}));
assertEquals(-1, StringUtils.indexOfAny(null, new String[] {null}));
}
public void testLastIndexOfAny_StringStringarray() {
assertEquals(-1, StringUtils.lastIndexOfAny(null, null));
assertEquals(-1, StringUtils.lastIndexOfAny(null, FOOBAR_SUB_ARRAY));
assertEquals(-1, StringUtils.lastIndexOfAny(FOOBAR, null));
assertEquals(3, StringUtils.lastIndexOfAny(FOOBAR, FOOBAR_SUB_ARRAY));
assertEquals(-1, StringUtils.lastIndexOfAny(FOOBAR, new String[0]));
assertEquals(-1, StringUtils.lastIndexOfAny(null, new String[0]));
assertEquals(-1, StringUtils.lastIndexOfAny("", new String[0]));
assertEquals(-1, StringUtils.lastIndexOfAny(FOOBAR, new String[] {"llll"}));
assertEquals(6, StringUtils.lastIndexOfAny(FOOBAR, new String[] {""}));
assertEquals(0, StringUtils.lastIndexOfAny("", new String[] {""}));
assertEquals(-1, StringUtils.lastIndexOfAny("", new String[] {"a"}));
assertEquals(-1, StringUtils.lastIndexOfAny("", new String[] {null}));
assertEquals(-1, StringUtils.lastIndexOfAny(FOOBAR, new String[] {null}));
assertEquals(-1, StringUtils.lastIndexOfAny(null, new String[] {null}));
}
//-----------------------------------------------------------------------
public void testIndexOfAny_StringChararray() {
assertEquals(-1, StringUtils.indexOfAny(null, (char[]) null));
assertEquals(-1, StringUtils.indexOfAny(null, new char[0]));
assertEquals(-1, StringUtils.indexOfAny(null, new char[] {'a','b'}));
assertEquals(-1, StringUtils.indexOfAny("", (char[]) null));
assertEquals(-1, StringUtils.indexOfAny("", new char[0]));
assertEquals(-1, StringUtils.indexOfAny("", new char[] {'a','b'}));
assertEquals(-1, StringUtils.indexOfAny("zzabyycdxx", (char[]) null));
assertEquals(-1, StringUtils.indexOfAny("zzabyycdxx", new char[0]));
assertEquals(0, StringUtils.indexOfAny("zzabyycdxx", new char[] {'z','a'}));
assertEquals(3, StringUtils.indexOfAny("zzabyycdxx", new char[] {'b','y'}));
assertEquals(-1, StringUtils.indexOfAny("ab", new char[] {'z'}));
}
public void testIndexOfAny_StringString() {
assertEquals(-1, StringUtils.indexOfAny(null, (String) null));
assertEquals(-1, StringUtils.indexOfAny(null, ""));
assertEquals(-1, StringUtils.indexOfAny(null, "ab"));
assertEquals(-1, StringUtils.indexOfAny("", (String) null));
assertEquals(-1, StringUtils.indexOfAny("", ""));
assertEquals(-1, StringUtils.indexOfAny("", "ab"));
assertEquals(-1, StringUtils.indexOfAny("zzabyycdxx", (String) null));
assertEquals(-1, StringUtils.indexOfAny("zzabyycdxx", ""));
assertEquals(0, StringUtils.indexOfAny("zzabyycdxx", "za"));
assertEquals(3, StringUtils.indexOfAny("zzabyycdxx", "by"));
assertEquals(-1, StringUtils.indexOfAny("ab", "z"));
}
//-----------------------------------------------------------------------
public void testContainsAny_StringChararray() {
assertFalse(StringUtils.containsAny(null, (char[]) null));
assertFalse(StringUtils.containsAny(null, new char[0]));
assertFalse(StringUtils.containsAny(null, new char[] {'a','b'}));
assertFalse(StringUtils.containsAny("", (char[]) null));
assertFalse(StringUtils.containsAny("", new char[0]));
assertFalse(StringUtils.containsAny("", new char[] {'a','b'}));
assertFalse(StringUtils.containsAny("zzabyycdxx", (char[]) null));
assertFalse(StringUtils.containsAny("zzabyycdxx", new char[0]));
assertTrue(StringUtils.containsAny("zzabyycdxx", new char[] {'z','a'}));
assertTrue(StringUtils.containsAny("zzabyycdxx", new char[] {'b','y'}));
assertFalse(StringUtils.containsAny("ab", new char[] {'z'}));
}
public void testContainsAny_StringString() {
assertFalse(StringUtils.containsAny(null, (String) null));
assertFalse(StringUtils.containsAny(null, ""));
assertFalse(StringUtils.containsAny(null, "ab"));
assertFalse(StringUtils.containsAny("", (String) null));
assertFalse(StringUtils.containsAny("", ""));
assertFalse(StringUtils.containsAny("", "ab"));
assertFalse(StringUtils.containsAny("zzabyycdxx", (String) null));
assertFalse(StringUtils.containsAny("zzabyycdxx", ""));
assertTrue(StringUtils.containsAny("zzabyycdxx", "za"));
assertTrue(StringUtils.containsAny("zzabyycdxx", "by"));
assertFalse(StringUtils.containsAny("ab", "z"));
}
//-----------------------------------------------------------------------
public void testIndexOfAnyBut_StringChararray() {
assertEquals(-1, StringUtils.indexOfAnyBut(null, (char[]) null));
assertEquals(-1, StringUtils.indexOfAnyBut(null, new char[0]));
assertEquals(-1, StringUtils.indexOfAnyBut(null, new char[] {'a','b'}));
assertEquals(-1, StringUtils.indexOfAnyBut("", (char[]) null));
assertEquals(-1, StringUtils.indexOfAnyBut("", new char[0]));
assertEquals(-1, StringUtils.indexOfAnyBut("", new char[] {'a','b'}));
assertEquals(-1, StringUtils.indexOfAnyBut("zzabyycdxx", (char[]) null));
assertEquals(-1, StringUtils.indexOfAnyBut("zzabyycdxx", new char[0]));
assertEquals(3, StringUtils.indexOfAnyBut("zzabyycdxx", new char[] {'z','a'}));
assertEquals(0, StringUtils.indexOfAnyBut("zzabyycdxx", new char[] {'b','y'}));
assertEquals(0, StringUtils.indexOfAnyBut("ab", new char[] {'z'}));
}
public void testIndexOfAnyBut_StringString() {
assertEquals(-1, StringUtils.indexOfAnyBut(null, (String) null));
assertEquals(-1, StringUtils.indexOfAnyBut(null, ""));
assertEquals(-1, StringUtils.indexOfAnyBut(null, "ab"));
assertEquals(-1, StringUtils.indexOfAnyBut("", (String) null));
assertEquals(-1, StringUtils.indexOfAnyBut("", ""));
assertEquals(-1, StringUtils.indexOfAnyBut("", "ab"));
assertEquals(-1, StringUtils.indexOfAnyBut("zzabyycdxx", (String) null));
assertEquals(-1, StringUtils.indexOfAnyBut("zzabyycdxx", ""));
assertEquals(3, StringUtils.indexOfAnyBut("zzabyycdxx", "za"));
assertEquals(0, StringUtils.indexOfAnyBut("zzabyycdxx", "by"));
assertEquals(0, StringUtils.indexOfAnyBut("ab", "z"));
}
//-----------------------------------------------------------------------
public void testContainsOnly_String() {
String str1 = "a";
String str2 = "b";
String str3 = "ab";
String chars1= "b";
String chars2= "a";
String chars3= "ab";
assertEquals(false, StringUtils.containsOnly(null, (String) null));
assertEquals(false, StringUtils.containsOnly("", (String) null));
assertEquals(false, StringUtils.containsOnly(null, ""));
assertEquals(false, StringUtils.containsOnly(str1, ""));
assertEquals(true, StringUtils.containsOnly("", ""));
assertEquals(true, StringUtils.containsOnly("", chars1));
assertEquals(false, StringUtils.containsOnly(str1, chars1));
assertEquals(true, StringUtils.containsOnly(str1, chars2));
assertEquals(true, StringUtils.containsOnly(str1, chars3));
assertEquals(true, StringUtils.containsOnly(str2, chars1));
assertEquals(false, StringUtils.containsOnly(str2, chars2));
assertEquals(true, StringUtils.containsOnly(str2, chars3));
assertEquals(false, StringUtils.containsOnly(str3, chars1));
assertEquals(false, StringUtils.containsOnly(str3, chars2));
assertEquals(true, StringUtils.containsOnly(str3, chars3));
}
public void testContainsOnly_Chararray() {
String str1 = "a";
String str2 = "b";
String str3 = "ab";
char[] chars1= {'b'};
char[] chars2= {'a'};
char[] chars3= {'a', 'b'};
char[] emptyChars = new char[0];
assertEquals(false, StringUtils.containsOnly(null, (char[]) null));
assertEquals(false, StringUtils.containsOnly("", (char[]) null));
assertEquals(false, StringUtils.containsOnly(null, emptyChars));
assertEquals(false, StringUtils.containsOnly(str1, emptyChars));
assertEquals(true, StringUtils.containsOnly("", emptyChars));
assertEquals(true, StringUtils.containsOnly("", chars1));
assertEquals(false, StringUtils.containsOnly(str1, chars1));
assertEquals(true, StringUtils.containsOnly(str1, chars2));
assertEquals(true, StringUtils.containsOnly(str1, chars3));
assertEquals(true, StringUtils.containsOnly(str2, chars1));
assertEquals(false, StringUtils.containsOnly(str2, chars2));
assertEquals(true, StringUtils.containsOnly(str2, chars3));
assertEquals(false, StringUtils.containsOnly(str3, chars1));
assertEquals(false, StringUtils.containsOnly(str3, chars2));
assertEquals(true, StringUtils.containsOnly(str3, chars3));
}
public void testContainsNone_String() {
String str1 = "a";
String str2 = "b";
String str3 = "ab.";
String chars1= "b";
String chars2= ".";
String chars3= "cd";
assertEquals(true, StringUtils.containsNone(null, (String) null));
assertEquals(true, StringUtils.containsNone("", (String) null));
assertEquals(true, StringUtils.containsNone(null, ""));
assertEquals(true, StringUtils.containsNone(str1, ""));
assertEquals(true, StringUtils.containsNone("", ""));
assertEquals(true, StringUtils.containsNone("", chars1));
assertEquals(true, StringUtils.containsNone(str1, chars1));
assertEquals(true, StringUtils.containsNone(str1, chars2));
assertEquals(true, StringUtils.containsNone(str1, chars3));
assertEquals(false, StringUtils.containsNone(str2, chars1));
assertEquals(true, StringUtils.containsNone(str2, chars2));
assertEquals(true, StringUtils.containsNone(str2, chars3));
assertEquals(false, StringUtils.containsNone(str3, chars1));
assertEquals(false, StringUtils.containsNone(str3, chars2));
assertEquals(true, StringUtils.containsNone(str3, chars3));
}
public void testContainsNone_Chararray() {
String str1 = "a";
String str2 = "b";
String str3 = "ab.";
char[] chars1= {'b'};
char[] chars2= {'.'};
char[] chars3= {'c', 'd'};
char[] emptyChars = new char[0];
assertEquals(true, StringUtils.containsNone(null, (char[]) null));
assertEquals(true, StringUtils.containsNone("", (char[]) null));
assertEquals(true, StringUtils.containsNone(null, emptyChars));
assertEquals(true, StringUtils.containsNone(str1, emptyChars));
assertEquals(true, StringUtils.containsNone("", emptyChars));
assertEquals(true, StringUtils.containsNone("", chars1));
assertEquals(true, StringUtils.containsNone(str1, chars1));
assertEquals(true, StringUtils.containsNone(str1, chars2));
assertEquals(true, StringUtils.containsNone(str1, chars3));
assertEquals(false, StringUtils.containsNone(str2, chars1));
assertEquals(true, StringUtils.containsNone(str2, chars2));
assertEquals(true, StringUtils.containsNone(str2, chars3));
assertEquals(false, StringUtils.containsNone(str3, chars1));
assertEquals(false, StringUtils.containsNone(str3, chars2));
assertEquals(true, StringUtils.containsNone(str3, chars3));
}
/**
* See http://java.sun.com/developer/technicalArticles/Intl/Supplementary/
*/
public void testContainsStringWithSupplementaryChars() {
assertEquals(true, StringUtils.contains(CharU20000 + CharU20001, CharU20000));
assertEquals(true, StringUtils.contains(CharU20000 + CharU20001, CharU20001));
assertEquals(true, StringUtils.contains(CharU20000, CharU20000));
assertEquals(false, StringUtils.contains(CharU20000, CharU20001));
}
/**
* See http://java.sun.com/developer/technicalArticles/Intl/Supplementary/
*/
public void testContainsAnyStringWithSupplementaryChars() {
assertEquals(true, StringUtils.containsAny(CharU20000 + CharU20001, CharU20000));
assertEquals(true, StringUtils.containsAny(CharU20000 + CharU20001, CharU20001));
assertEquals(true, StringUtils.containsAny(CharU20000, CharU20000));
// Sanity check:
assertEquals(-1, CharU20000.indexOf(CharU20001));
assertEquals(0, CharU20000.indexOf(CharU20001.charAt(0)));
assertEquals(-1, CharU20000.indexOf(CharU20001.charAt(1)));
// Test:
assertEquals(false, StringUtils.containsAny(CharU20000, CharU20001));
assertEquals(false, StringUtils.containsAny(CharU20001, CharU20000));
}
/**
* See http://java.sun.com/developer/technicalArticles/Intl/Supplementary/
*/
public void testContainsAnyCharArrayWithSupplementaryChars() {
assertEquals(true, StringUtils.containsAny(CharU20000 + CharU20001, CharU20000.toCharArray()));
assertEquals(true, StringUtils.containsAny(CharU20000 + CharU20001, CharU20001.toCharArray()));
assertEquals(true, StringUtils.containsAny(CharU20000, CharU20000.toCharArray()));
// Sanity check:
assertEquals(-1, CharU20000.indexOf(CharU20001));
assertEquals(0, CharU20000.indexOf(CharU20001.charAt(0)));
assertEquals(-1, CharU20000.indexOf(CharU20001.charAt(1)));
// Test:
assertEquals(false, StringUtils.containsAny(CharU20000, CharU20001.toCharArray()));
assertEquals(false, StringUtils.containsAny(CharU20001, CharU20000.toCharArray()));
}
} | // You are a professional Java test case writer, please create a test case named `testContainsAnyCharArrayWithSupplementaryChars` for the issue `Lang-LANG-607`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Lang-LANG-607
//
// ## Issue-Title:
// StringUtils methods do not handle Unicode 2.0+ supplementary characters correctly.
//
// ## Issue-Description:
//
// StringUtils.containsAny methods incorrectly matches Unicode 2.0+ supplementary characters.
//
//
// For example, define a test fixture to be the Unicode character U+20000 where U+20000 is written in Java source as "\uD840\uDC00"
//
//
// private static final String CharU20000 = "\uD840\uDC00";
//
// private static final String CharU20001 = "\uD840\uDC01";
//
//
// You can see Unicode supplementary characters correctly implemented in the JRE call:
//
//
// assertEquals(-1, CharU20000.indexOf(CharU20001));
//
//
// But this is broken:
//
//
// assertEquals(false, StringUtils.containsAny(CharU20000, CharU20001));
//
// assertEquals(false, StringUtils.containsAny(CharU20001, CharU20000));
//
//
// This is fine:
//
//
// assertEquals(true, StringUtils.contains(CharU20000 + CharU20001, CharU20000));
//
// assertEquals(true, StringUtils.contains(CharU20000 + CharU20001, CharU20001));
//
// assertEquals(true, StringUtils.contains(CharU20000, CharU20000));
//
// assertEquals(false, StringUtils.contains(CharU20000, CharU20001));
//
//
// because the method calls the JRE to perform the match.
//
//
// More than you want to know:
//
//
// * <http://java.sun.com/developer/technicalArticles/Intl/Supplementary/>
//
//
//
//
//
public void testContainsAnyCharArrayWithSupplementaryChars() {
| 697 | /**
* See http://java.sun.com/developer/technicalArticles/Intl/Supplementary/
*/ | 31 | 686 | src/test/java/org/apache/commons/lang3/StringUtilsEqualsIndexOfTest.java | src/test/java | ```markdown
## Issue-ID: Lang-LANG-607
## Issue-Title:
StringUtils methods do not handle Unicode 2.0+ supplementary characters correctly.
## Issue-Description:
StringUtils.containsAny methods incorrectly matches Unicode 2.0+ supplementary characters.
For example, define a test fixture to be the Unicode character U+20000 where U+20000 is written in Java source as "\uD840\uDC00"
private static final String CharU20000 = "\uD840\uDC00";
private static final String CharU20001 = "\uD840\uDC01";
You can see Unicode supplementary characters correctly implemented in the JRE call:
assertEquals(-1, CharU20000.indexOf(CharU20001));
But this is broken:
assertEquals(false, StringUtils.containsAny(CharU20000, CharU20001));
assertEquals(false, StringUtils.containsAny(CharU20001, CharU20000));
This is fine:
assertEquals(true, StringUtils.contains(CharU20000 + CharU20001, CharU20000));
assertEquals(true, StringUtils.contains(CharU20000 + CharU20001, CharU20001));
assertEquals(true, StringUtils.contains(CharU20000, CharU20000));
assertEquals(false, StringUtils.contains(CharU20000, CharU20001));
because the method calls the JRE to perform the match.
More than you want to know:
* <http://java.sun.com/developer/technicalArticles/Intl/Supplementary/>
```
You are a professional Java test case writer, please create a test case named `testContainsAnyCharArrayWithSupplementaryChars` for the issue `Lang-LANG-607`, utilizing the provided issue report information and the following function signature.
```java
public void testContainsAnyCharArrayWithSupplementaryChars() {
```
| 686 | [
"org.apache.commons.lang3.StringUtils"
] | 7beb15d4366eb6e820d91951d7e7606344d29a48ec64fe37201adcd28ca62008 | public void testContainsAnyCharArrayWithSupplementaryChars() | // You are a professional Java test case writer, please create a test case named `testContainsAnyCharArrayWithSupplementaryChars` for the issue `Lang-LANG-607`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Lang-LANG-607
//
// ## Issue-Title:
// StringUtils methods do not handle Unicode 2.0+ supplementary characters correctly.
//
// ## Issue-Description:
//
// StringUtils.containsAny methods incorrectly matches Unicode 2.0+ supplementary characters.
//
//
// For example, define a test fixture to be the Unicode character U+20000 where U+20000 is written in Java source as "\uD840\uDC00"
//
//
// private static final String CharU20000 = "\uD840\uDC00";
//
// private static final String CharU20001 = "\uD840\uDC01";
//
//
// You can see Unicode supplementary characters correctly implemented in the JRE call:
//
//
// assertEquals(-1, CharU20000.indexOf(CharU20001));
//
//
// But this is broken:
//
//
// assertEquals(false, StringUtils.containsAny(CharU20000, CharU20001));
//
// assertEquals(false, StringUtils.containsAny(CharU20001, CharU20000));
//
//
// This is fine:
//
//
// assertEquals(true, StringUtils.contains(CharU20000 + CharU20001, CharU20000));
//
// assertEquals(true, StringUtils.contains(CharU20000 + CharU20001, CharU20001));
//
// assertEquals(true, StringUtils.contains(CharU20000, CharU20000));
//
// assertEquals(false, StringUtils.contains(CharU20000, CharU20001));
//
//
// because the method calls the JRE to perform the match.
//
//
// More than you want to know:
//
//
// * <http://java.sun.com/developer/technicalArticles/Intl/Supplementary/>
//
//
//
//
//
| Lang | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.lang3;
import java.util.Locale;
import junit.framework.TestCase;
/**
* Unit tests {@link org.apache.commons.lang3.StringUtils} - Substring methods
*
* @author Apache Software Foundation
* @author <a href="mailto:[email protected]">Ringo De Smet</a>
* @author Phil Steitz
* @version $Id$
*/
public class StringUtilsEqualsIndexOfTest extends TestCase {
private static final String FOO = "foo";
private static final String BAR = "bar";
private static final String FOOBAR = "foobar";
private static final String[] FOOBAR_SUB_ARRAY = new String[] {"ob", "ba"};
/**
* Supplementary character U+20000
* See http://java.sun.com/developer/technicalArticles/Intl/Supplementary/
*/
private static final String CharU20000 = "\uD840\uDC00";
/**
* Supplementary character U+20001
* See http://java.sun.com/developer/technicalArticles/Intl/Supplementary/
*/
private static final String CharU20001 = "\uD840\uDC01";
public StringUtilsEqualsIndexOfTest(String name) {
super(name);
}
//-----------------------------------------------------------------------
public void testEquals() {
assertEquals(true, StringUtils.equals(null, null));
assertEquals(true, StringUtils.equals(FOO, FOO));
assertEquals(true, StringUtils.equals(FOO, new String(new char[] { 'f', 'o', 'o' })));
assertEquals(false, StringUtils.equals(FOO, new String(new char[] { 'f', 'O', 'O' })));
assertEquals(false, StringUtils.equals(FOO, BAR));
assertEquals(false, StringUtils.equals(FOO, null));
assertEquals(false, StringUtils.equals(null, FOO));
}
public void testEqualsIgnoreCase() {
assertEquals(true, StringUtils.equalsIgnoreCase(null, null));
assertEquals(true, StringUtils.equalsIgnoreCase(FOO, FOO));
assertEquals(true, StringUtils.equalsIgnoreCase(FOO, new String(new char[] { 'f', 'o', 'o' })));
assertEquals(true, StringUtils.equalsIgnoreCase(FOO, new String(new char[] { 'f', 'O', 'O' })));
assertEquals(false, StringUtils.equalsIgnoreCase(FOO, BAR));
assertEquals(false, StringUtils.equalsIgnoreCase(FOO, null));
assertEquals(false, StringUtils.equalsIgnoreCase(null, FOO));
}
//-----------------------------------------------------------------------
public void testIndexOf_char() {
assertEquals(-1, StringUtils.indexOf(null, ' '));
assertEquals(-1, StringUtils.indexOf("", ' '));
assertEquals(0, StringUtils.indexOf("aabaabaa", 'a'));
assertEquals(2, StringUtils.indexOf("aabaabaa", 'b'));
}
public void testIndexOf_charInt() {
assertEquals(-1, StringUtils.indexOf(null, ' ', 0));
assertEquals(-1, StringUtils.indexOf(null, ' ', -1));
assertEquals(-1, StringUtils.indexOf("", ' ', 0));
assertEquals(-1, StringUtils.indexOf("", ' ', -1));
assertEquals(0, StringUtils.indexOf("aabaabaa", 'a', 0));
assertEquals(2, StringUtils.indexOf("aabaabaa", 'b', 0));
assertEquals(5, StringUtils.indexOf("aabaabaa", 'b', 3));
assertEquals(-1, StringUtils.indexOf("aabaabaa", 'b', 9));
assertEquals(2, StringUtils.indexOf("aabaabaa", 'b', -1));
}
public void testIndexOf_String() {
assertEquals(-1, StringUtils.indexOf(null, null));
assertEquals(-1, StringUtils.indexOf("", null));
assertEquals(0, StringUtils.indexOf("", ""));
assertEquals(0, StringUtils.indexOf("aabaabaa", "a"));
assertEquals(2, StringUtils.indexOf("aabaabaa", "b"));
assertEquals(1, StringUtils.indexOf("aabaabaa", "ab"));
assertEquals(0, StringUtils.indexOf("aabaabaa", ""));
}
public void testIndexOfIgnoreCase_String() {
assertEquals(-1, StringUtils.indexOfIgnoreCase(null, null));
assertEquals(-1, StringUtils.indexOfIgnoreCase(null, ""));
assertEquals(-1, StringUtils.indexOfIgnoreCase("", null));
assertEquals(0, StringUtils.indexOfIgnoreCase("", ""));
assertEquals(0, StringUtils.indexOfIgnoreCase("aabaabaa", "a"));
assertEquals(0, StringUtils.indexOfIgnoreCase("aabaabaa", "A"));
assertEquals(2, StringUtils.indexOfIgnoreCase("aabaabaa", "b"));
assertEquals(2, StringUtils.indexOfIgnoreCase("aabaabaa", "B"));
assertEquals(1, StringUtils.indexOfIgnoreCase("aabaabaa", "ab"));
assertEquals(1, StringUtils.indexOfIgnoreCase("aabaabaa", "AB"));
assertEquals(0, StringUtils.indexOfIgnoreCase("aabaabaa", ""));
}
public void testIndexOfIgnoreCase_StringInt() {
assertEquals(1, StringUtils.indexOfIgnoreCase("aabaabaa", "AB", -1));
assertEquals(1, StringUtils.indexOfIgnoreCase("aabaabaa", "AB", 0));
assertEquals(1, StringUtils.indexOfIgnoreCase("aabaabaa", "AB", 1));
assertEquals(4, StringUtils.indexOfIgnoreCase("aabaabaa", "AB", 2));
assertEquals(4, StringUtils.indexOfIgnoreCase("aabaabaa", "AB", 3));
assertEquals(4, StringUtils.indexOfIgnoreCase("aabaabaa", "AB", 4));
assertEquals(-1, StringUtils.indexOfIgnoreCase("aabaabaa", "AB", 5));
assertEquals(-1, StringUtils.indexOfIgnoreCase("aabaabaa", "AB", 6));
assertEquals(-1, StringUtils.indexOfIgnoreCase("aabaabaa", "AB", 7));
assertEquals(-1, StringUtils.indexOfIgnoreCase("aabaabaa", "AB", 8));
assertEquals(1, StringUtils.indexOfIgnoreCase("aab", "AB", 1));
assertEquals(5, StringUtils.indexOfIgnoreCase("aabaabaa", "", 5));
assertEquals(-1, StringUtils.indexOfIgnoreCase("ab", "AAB", 0));
assertEquals(-1, StringUtils.indexOfIgnoreCase("aab", "AAB", 1));
}
public void testOrdinalIndexOf() {
assertEquals(-1, StringUtils.ordinalIndexOf(null, null, Integer.MIN_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("", null, Integer.MIN_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("", "", Integer.MIN_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "a", Integer.MIN_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "b", Integer.MIN_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "ab", Integer.MIN_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "", Integer.MIN_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf(null, null, -1));
assertEquals(-1, StringUtils.ordinalIndexOf("", null, -1));
assertEquals(-1, StringUtils.ordinalIndexOf("", "", -1));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "a", -1));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "b", -1));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "ab", -1));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "", -1));
assertEquals(-1, StringUtils.ordinalIndexOf(null, null, 0));
assertEquals(-1, StringUtils.ordinalIndexOf("", null, 0));
assertEquals(-1, StringUtils.ordinalIndexOf("", "", 0));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "a", 0));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "b", 0));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "ab", 0));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "", 0));
assertEquals(-1, StringUtils.ordinalIndexOf(null, null, 1));
assertEquals(-1, StringUtils.ordinalIndexOf("", null, 1));
assertEquals(0, StringUtils.ordinalIndexOf("", "", 1));
assertEquals(0, StringUtils.ordinalIndexOf("aabaabaa", "a", 1));
assertEquals(2, StringUtils.ordinalIndexOf("aabaabaa", "b", 1));
assertEquals(1, StringUtils.ordinalIndexOf("aabaabaa", "ab", 1));
assertEquals(0, StringUtils.ordinalIndexOf("aabaabaa", "", 1));
assertEquals(-1, StringUtils.ordinalIndexOf(null, null, 2));
assertEquals(-1, StringUtils.ordinalIndexOf("", null, 2));
assertEquals(0, StringUtils.ordinalIndexOf("", "", 2));
assertEquals(1, StringUtils.ordinalIndexOf("aabaabaa", "a", 2));
assertEquals(5, StringUtils.ordinalIndexOf("aabaabaa", "b", 2));
assertEquals(4, StringUtils.ordinalIndexOf("aabaabaa", "ab", 2));
assertEquals(0, StringUtils.ordinalIndexOf("aabaabaa", "", 2));
assertEquals(-1, StringUtils.ordinalIndexOf(null, null, Integer.MAX_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("", null, Integer.MAX_VALUE));
assertEquals(0, StringUtils.ordinalIndexOf("", "", Integer.MAX_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "a", Integer.MAX_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "b", Integer.MAX_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("aabaabaa", "ab", Integer.MAX_VALUE));
assertEquals(0, StringUtils.ordinalIndexOf("aabaabaa", "", Integer.MAX_VALUE));
assertEquals(-1, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 0));
assertEquals(0, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 1));
assertEquals(1, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 2));
assertEquals(2, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 3));
assertEquals(3, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 4));
assertEquals(4, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 5));
assertEquals(5, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 6));
assertEquals(6, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 7));
assertEquals(7, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 8));
assertEquals(8, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 9));
assertEquals(-1, StringUtils.ordinalIndexOf("aaaaaaaaa", "a", 10));
}
public void testIndexOf_StringInt() {
assertEquals(-1, StringUtils.indexOf(null, null, 0));
assertEquals(-1, StringUtils.indexOf(null, null, -1));
assertEquals(-1, StringUtils.indexOf(null, "", 0));
assertEquals(-1, StringUtils.indexOf(null, "", -1));
assertEquals(-1, StringUtils.indexOf("", null, 0));
assertEquals(-1, StringUtils.indexOf("", null, -1));
assertEquals(0, StringUtils.indexOf("", "", 0));
assertEquals(0, StringUtils.indexOf("", "", -1));
assertEquals(0, StringUtils.indexOf("", "", 9));
assertEquals(0, StringUtils.indexOf("abc", "", 0));
assertEquals(0, StringUtils.indexOf("abc", "", -1));
assertEquals(3, StringUtils.indexOf("abc", "", 9));
assertEquals(3, StringUtils.indexOf("abc", "", 3));
assertEquals(0, StringUtils.indexOf("aabaabaa", "a", 0));
assertEquals(2, StringUtils.indexOf("aabaabaa", "b", 0));
assertEquals(1, StringUtils.indexOf("aabaabaa", "ab", 0));
assertEquals(5, StringUtils.indexOf("aabaabaa", "b", 3));
assertEquals(-1, StringUtils.indexOf("aabaabaa", "b", 9));
assertEquals(2, StringUtils.indexOf("aabaabaa", "b", -1));
assertEquals(2,StringUtils.indexOf("aabaabaa", "", 2));
}
//-----------------------------------------------------------------------
public void testLastIndexOf_char() {
assertEquals(-1, StringUtils.lastIndexOf(null, ' '));
assertEquals(-1, StringUtils.lastIndexOf("", ' '));
assertEquals(7, StringUtils.lastIndexOf("aabaabaa", 'a'));
assertEquals(5, StringUtils.lastIndexOf("aabaabaa", 'b'));
}
public void testLastIndexOf_charInt() {
assertEquals(-1, StringUtils.lastIndexOf(null, ' ', 0));
assertEquals(-1, StringUtils.lastIndexOf(null, ' ', -1));
assertEquals(-1, StringUtils.lastIndexOf("", ' ', 0));
assertEquals(-1, StringUtils.lastIndexOf("", ' ', -1));
assertEquals(7, StringUtils.lastIndexOf("aabaabaa", 'a', 8));
assertEquals(5, StringUtils.lastIndexOf("aabaabaa", 'b', 8));
assertEquals(2, StringUtils.lastIndexOf("aabaabaa", 'b', 3));
assertEquals(5, StringUtils.lastIndexOf("aabaabaa", 'b', 9));
assertEquals(-1, StringUtils.lastIndexOf("aabaabaa", 'b', -1));
assertEquals(0, StringUtils.lastIndexOf("aabaabaa", 'a', 0));
}
public void testLastIndexOf_String() {
assertEquals(-1, StringUtils.lastIndexOf(null, null));
assertEquals(-1, StringUtils.lastIndexOf("", null));
assertEquals(-1, StringUtils.lastIndexOf("", "a"));
assertEquals(0, StringUtils.lastIndexOf("", ""));
assertEquals(8, StringUtils.lastIndexOf("aabaabaa", ""));
assertEquals(7, StringUtils.lastIndexOf("aabaabaa", "a"));
assertEquals(5, StringUtils.lastIndexOf("aabaabaa", "b"));
assertEquals(4, StringUtils.lastIndexOf("aabaabaa", "ab"));
}
public void testLastOrdinalIndexOf() {
assertEquals(-1, StringUtils.lastOrdinalIndexOf(null, "*", 42) );
assertEquals(-1, StringUtils.lastOrdinalIndexOf("*", null, 42) );
assertEquals(0, StringUtils.lastOrdinalIndexOf("", "", 42) );
assertEquals(7, StringUtils.lastOrdinalIndexOf("aabaabaa", "a", 1) );
assertEquals(6, StringUtils.lastOrdinalIndexOf("aabaabaa", "a", 2) );
assertEquals(5, StringUtils.lastOrdinalIndexOf("aabaabaa", "b", 1) );
assertEquals(2, StringUtils.lastOrdinalIndexOf("aabaabaa", "b", 2) );
assertEquals(4, StringUtils.lastOrdinalIndexOf("aabaabaa", "ab", 1) );
assertEquals(1, StringUtils.lastOrdinalIndexOf("aabaabaa", "ab", 2) );
assertEquals(8, StringUtils.lastOrdinalIndexOf("aabaabaa", "", 1) );
assertEquals(8, StringUtils.lastOrdinalIndexOf("aabaabaa", "", 2) );
}
public void testLastIndexOf_StringInt() {
assertEquals(-1, StringUtils.lastIndexOf(null, null, 0));
assertEquals(-1, StringUtils.lastIndexOf(null, null, -1));
assertEquals(-1, StringUtils.lastIndexOf(null, "", 0));
assertEquals(-1, StringUtils.lastIndexOf(null, "", -1));
assertEquals(-1, StringUtils.lastIndexOf("", null, 0));
assertEquals(-1, StringUtils.lastIndexOf("", null, -1));
assertEquals(0, StringUtils.lastIndexOf("", "", 0));
assertEquals(-1, StringUtils.lastIndexOf("", "", -1));
assertEquals(0, StringUtils.lastIndexOf("", "", 9));
assertEquals(0, StringUtils.lastIndexOf("abc", "", 0));
assertEquals(-1, StringUtils.lastIndexOf("abc", "", -1));
assertEquals(3, StringUtils.lastIndexOf("abc", "", 9));
assertEquals(7, StringUtils.lastIndexOf("aabaabaa", "a", 8));
assertEquals(5, StringUtils.lastIndexOf("aabaabaa", "b", 8));
assertEquals(4, StringUtils.lastIndexOf("aabaabaa", "ab", 8));
assertEquals(2, StringUtils.lastIndexOf("aabaabaa", "b", 3));
assertEquals(5, StringUtils.lastIndexOf("aabaabaa", "b", 9));
assertEquals(-1, StringUtils.lastIndexOf("aabaabaa", "b", -1));
assertEquals(-1, StringUtils.lastIndexOf("aabaabaa", "b", 0));
assertEquals(0, StringUtils.lastIndexOf("aabaabaa", "a", 0));
}
public void testLastIndexOfIgnoreCase_String() {
assertEquals(-1, StringUtils.lastIndexOfIgnoreCase(null, null));
assertEquals(-1, StringUtils.lastIndexOfIgnoreCase("", null));
assertEquals(-1, StringUtils.lastIndexOfIgnoreCase(null, ""));
assertEquals(-1, StringUtils.lastIndexOfIgnoreCase("", "a"));
assertEquals(0, StringUtils.lastIndexOfIgnoreCase("", ""));
assertEquals(8, StringUtils.lastIndexOfIgnoreCase("aabaabaa", ""));
assertEquals(7, StringUtils.lastIndexOfIgnoreCase("aabaabaa", "a"));
assertEquals(7, StringUtils.lastIndexOfIgnoreCase("aabaabaa", "A"));
assertEquals(5, StringUtils.lastIndexOfIgnoreCase("aabaabaa", "b"));
assertEquals(5, StringUtils.lastIndexOfIgnoreCase("aabaabaa", "B"));
assertEquals(4, StringUtils.lastIndexOfIgnoreCase("aabaabaa", "ab"));
assertEquals(4, StringUtils.lastIndexOfIgnoreCase("aabaabaa", "AB"));
assertEquals(-1, StringUtils.lastIndexOfIgnoreCase("ab", "AAB"));
assertEquals(0, StringUtils.lastIndexOfIgnoreCase("aab", "AAB"));
}
public void testLastIndexOfIgnoreCase_StringInt() {
assertEquals(-1, StringUtils.lastIndexOfIgnoreCase(null, null, 0));
assertEquals(-1, StringUtils.lastIndexOfIgnoreCase(null, null, -1));
assertEquals(-1, StringUtils.lastIndexOfIgnoreCase(null, "", 0));
assertEquals(-1, StringUtils.lastIndexOfIgnoreCase(null, "", -1));
assertEquals(-1, StringUtils.lastIndexOfIgnoreCase("", null, 0));
assertEquals(-1, StringUtils.lastIndexOfIgnoreCase("", null, -1));
assertEquals(0, StringUtils.lastIndexOfIgnoreCase("", "", 0));
assertEquals(-1, StringUtils.lastIndexOfIgnoreCase("", "", -1));
assertEquals(0, StringUtils.lastIndexOfIgnoreCase("", "", 9));
assertEquals(0, StringUtils.lastIndexOfIgnoreCase("abc", "", 0));
assertEquals(-1, StringUtils.lastIndexOfIgnoreCase("abc", "", -1));
assertEquals(3, StringUtils.lastIndexOfIgnoreCase("abc", "", 9));
assertEquals(7, StringUtils.lastIndexOfIgnoreCase("aabaabaa", "A", 8));
assertEquals(5, StringUtils.lastIndexOfIgnoreCase("aabaabaa", "B", 8));
assertEquals(4, StringUtils.lastIndexOfIgnoreCase("aabaabaa", "AB", 8));
assertEquals(2, StringUtils.lastIndexOfIgnoreCase("aabaabaa", "B", 3));
assertEquals(5, StringUtils.lastIndexOfIgnoreCase("aabaabaa", "B", 9));
assertEquals(-1, StringUtils.lastIndexOfIgnoreCase("aabaabaa", "B", -1));
assertEquals(-1, StringUtils.lastIndexOfIgnoreCase("aabaabaa", "B", 0));
assertEquals(0, StringUtils.lastIndexOfIgnoreCase("aabaabaa", "A", 0));
assertEquals(1, StringUtils.lastIndexOfIgnoreCase("aab", "AB", 1));
}
//-----------------------------------------------------------------------
public void testContainsChar() {
assertEquals(false, StringUtils.contains(null, ' '));
assertEquals(false, StringUtils.contains("", ' '));
assertEquals(false, StringUtils.contains("",null));
assertEquals(false, StringUtils.contains(null,null));
assertEquals(true, StringUtils.contains("abc", 'a'));
assertEquals(true, StringUtils.contains("abc", 'b'));
assertEquals(true, StringUtils.contains("abc", 'c'));
assertEquals(false, StringUtils.contains("abc", 'z'));
}
public void testContainsString() {
assertEquals(false, StringUtils.contains(null, null));
assertEquals(false, StringUtils.contains(null, ""));
assertEquals(false, StringUtils.contains(null, "a"));
assertEquals(false, StringUtils.contains("", null));
assertEquals(true, StringUtils.contains("", ""));
assertEquals(false, StringUtils.contains("", "a"));
assertEquals(true, StringUtils.contains("abc", "a"));
assertEquals(true, StringUtils.contains("abc", "b"));
assertEquals(true, StringUtils.contains("abc", "c"));
assertEquals(true, StringUtils.contains("abc", "abc"));
assertEquals(false, StringUtils.contains("abc", "z"));
}
public void testContainsIgnoreCase_StringString() {
assertFalse(StringUtils.containsIgnoreCase(null, null));
// Null tests
assertFalse(StringUtils.containsIgnoreCase(null, ""));
assertFalse(StringUtils.containsIgnoreCase(null, "a"));
assertFalse(StringUtils.containsIgnoreCase(null, "abc"));
assertFalse(StringUtils.containsIgnoreCase("", null));
assertFalse(StringUtils.containsIgnoreCase("a", null));
assertFalse(StringUtils.containsIgnoreCase("abc", null));
// Match len = 0
assertTrue(StringUtils.containsIgnoreCase("", ""));
assertTrue(StringUtils.containsIgnoreCase("a", ""));
assertTrue(StringUtils.containsIgnoreCase("abc", ""));
// Match len = 1
assertFalse(StringUtils.containsIgnoreCase("", "a"));
assertTrue(StringUtils.containsIgnoreCase("a", "a"));
assertTrue(StringUtils.containsIgnoreCase("abc", "a"));
assertFalse(StringUtils.containsIgnoreCase("", "A"));
assertTrue(StringUtils.containsIgnoreCase("a", "A"));
assertTrue(StringUtils.containsIgnoreCase("abc", "A"));
// Match len > 1
assertFalse(StringUtils.containsIgnoreCase("", "abc"));
assertFalse(StringUtils.containsIgnoreCase("a", "abc"));
assertTrue(StringUtils.containsIgnoreCase("xabcz", "abc"));
assertFalse(StringUtils.containsIgnoreCase("", "ABC"));
assertFalse(StringUtils.containsIgnoreCase("a", "ABC"));
assertTrue(StringUtils.containsIgnoreCase("xabcz", "ABC"));
}
public void testContainsIgnoreCase_LocaleIndependence() {
Locale orig = Locale.getDefault();
Locale[] locales = { Locale.ENGLISH, new Locale("tr"), Locale.getDefault() };
String[][] tdata = {
{ "i", "I" },
{ "I", "i" },
{ "\u03C2", "\u03C3" },
{ "\u03A3", "\u03C2" },
{ "\u03A3", "\u03C3" },
};
String[][] fdata = {
{ "\u00DF", "SS" },
};
try {
for (int i = 0; i < locales.length; i++) {
Locale.setDefault(locales[i]);
for (int j = 0; j < tdata.length; j++) {
assertTrue(Locale.getDefault() + ": " + j + " " + tdata[j][0] + " " + tdata[j][1], StringUtils
.containsIgnoreCase(tdata[j][0], tdata[j][1]));
}
for (int j = 0; j < fdata.length; j++) {
assertFalse(Locale.getDefault() + ": " + j + " " + fdata[j][0] + " " + fdata[j][1], StringUtils
.containsIgnoreCase(fdata[j][0], fdata[j][1]));
}
}
} finally {
Locale.setDefault(orig);
}
}
// -----------------------------------------------------------------------
public void testIndexOfAny_StringStringarray() {
assertEquals(-1, StringUtils.indexOfAny(null, (String[]) null));
assertEquals(-1, StringUtils.indexOfAny(null, FOOBAR_SUB_ARRAY));
assertEquals(-1, StringUtils.indexOfAny(FOOBAR, (String[]) null));
assertEquals(2, StringUtils.indexOfAny(FOOBAR, FOOBAR_SUB_ARRAY));
assertEquals(-1, StringUtils.indexOfAny(FOOBAR, new String[0]));
assertEquals(-1, StringUtils.indexOfAny(null, new String[0]));
assertEquals(-1, StringUtils.indexOfAny("", new String[0]));
assertEquals(-1, StringUtils.indexOfAny(FOOBAR, new String[] {"llll"}));
assertEquals(0, StringUtils.indexOfAny(FOOBAR, new String[] {""}));
assertEquals(0, StringUtils.indexOfAny("", new String[] {""}));
assertEquals(-1, StringUtils.indexOfAny("", new String[] {"a"}));
assertEquals(-1, StringUtils.indexOfAny("", new String[] {null}));
assertEquals(-1, StringUtils.indexOfAny(FOOBAR, new String[] {null}));
assertEquals(-1, StringUtils.indexOfAny(null, new String[] {null}));
}
public void testLastIndexOfAny_StringStringarray() {
assertEquals(-1, StringUtils.lastIndexOfAny(null, null));
assertEquals(-1, StringUtils.lastIndexOfAny(null, FOOBAR_SUB_ARRAY));
assertEquals(-1, StringUtils.lastIndexOfAny(FOOBAR, null));
assertEquals(3, StringUtils.lastIndexOfAny(FOOBAR, FOOBAR_SUB_ARRAY));
assertEquals(-1, StringUtils.lastIndexOfAny(FOOBAR, new String[0]));
assertEquals(-1, StringUtils.lastIndexOfAny(null, new String[0]));
assertEquals(-1, StringUtils.lastIndexOfAny("", new String[0]));
assertEquals(-1, StringUtils.lastIndexOfAny(FOOBAR, new String[] {"llll"}));
assertEquals(6, StringUtils.lastIndexOfAny(FOOBAR, new String[] {""}));
assertEquals(0, StringUtils.lastIndexOfAny("", new String[] {""}));
assertEquals(-1, StringUtils.lastIndexOfAny("", new String[] {"a"}));
assertEquals(-1, StringUtils.lastIndexOfAny("", new String[] {null}));
assertEquals(-1, StringUtils.lastIndexOfAny(FOOBAR, new String[] {null}));
assertEquals(-1, StringUtils.lastIndexOfAny(null, new String[] {null}));
}
//-----------------------------------------------------------------------
public void testIndexOfAny_StringChararray() {
assertEquals(-1, StringUtils.indexOfAny(null, (char[]) null));
assertEquals(-1, StringUtils.indexOfAny(null, new char[0]));
assertEquals(-1, StringUtils.indexOfAny(null, new char[] {'a','b'}));
assertEquals(-1, StringUtils.indexOfAny("", (char[]) null));
assertEquals(-1, StringUtils.indexOfAny("", new char[0]));
assertEquals(-1, StringUtils.indexOfAny("", new char[] {'a','b'}));
assertEquals(-1, StringUtils.indexOfAny("zzabyycdxx", (char[]) null));
assertEquals(-1, StringUtils.indexOfAny("zzabyycdxx", new char[0]));
assertEquals(0, StringUtils.indexOfAny("zzabyycdxx", new char[] {'z','a'}));
assertEquals(3, StringUtils.indexOfAny("zzabyycdxx", new char[] {'b','y'}));
assertEquals(-1, StringUtils.indexOfAny("ab", new char[] {'z'}));
}
public void testIndexOfAny_StringString() {
assertEquals(-1, StringUtils.indexOfAny(null, (String) null));
assertEquals(-1, StringUtils.indexOfAny(null, ""));
assertEquals(-1, StringUtils.indexOfAny(null, "ab"));
assertEquals(-1, StringUtils.indexOfAny("", (String) null));
assertEquals(-1, StringUtils.indexOfAny("", ""));
assertEquals(-1, StringUtils.indexOfAny("", "ab"));
assertEquals(-1, StringUtils.indexOfAny("zzabyycdxx", (String) null));
assertEquals(-1, StringUtils.indexOfAny("zzabyycdxx", ""));
assertEquals(0, StringUtils.indexOfAny("zzabyycdxx", "za"));
assertEquals(3, StringUtils.indexOfAny("zzabyycdxx", "by"));
assertEquals(-1, StringUtils.indexOfAny("ab", "z"));
}
//-----------------------------------------------------------------------
public void testContainsAny_StringChararray() {
assertFalse(StringUtils.containsAny(null, (char[]) null));
assertFalse(StringUtils.containsAny(null, new char[0]));
assertFalse(StringUtils.containsAny(null, new char[] {'a','b'}));
assertFalse(StringUtils.containsAny("", (char[]) null));
assertFalse(StringUtils.containsAny("", new char[0]));
assertFalse(StringUtils.containsAny("", new char[] {'a','b'}));
assertFalse(StringUtils.containsAny("zzabyycdxx", (char[]) null));
assertFalse(StringUtils.containsAny("zzabyycdxx", new char[0]));
assertTrue(StringUtils.containsAny("zzabyycdxx", new char[] {'z','a'}));
assertTrue(StringUtils.containsAny("zzabyycdxx", new char[] {'b','y'}));
assertFalse(StringUtils.containsAny("ab", new char[] {'z'}));
}
public void testContainsAny_StringString() {
assertFalse(StringUtils.containsAny(null, (String) null));
assertFalse(StringUtils.containsAny(null, ""));
assertFalse(StringUtils.containsAny(null, "ab"));
assertFalse(StringUtils.containsAny("", (String) null));
assertFalse(StringUtils.containsAny("", ""));
assertFalse(StringUtils.containsAny("", "ab"));
assertFalse(StringUtils.containsAny("zzabyycdxx", (String) null));
assertFalse(StringUtils.containsAny("zzabyycdxx", ""));
assertTrue(StringUtils.containsAny("zzabyycdxx", "za"));
assertTrue(StringUtils.containsAny("zzabyycdxx", "by"));
assertFalse(StringUtils.containsAny("ab", "z"));
}
//-----------------------------------------------------------------------
public void testIndexOfAnyBut_StringChararray() {
assertEquals(-1, StringUtils.indexOfAnyBut(null, (char[]) null));
assertEquals(-1, StringUtils.indexOfAnyBut(null, new char[0]));
assertEquals(-1, StringUtils.indexOfAnyBut(null, new char[] {'a','b'}));
assertEquals(-1, StringUtils.indexOfAnyBut("", (char[]) null));
assertEquals(-1, StringUtils.indexOfAnyBut("", new char[0]));
assertEquals(-1, StringUtils.indexOfAnyBut("", new char[] {'a','b'}));
assertEquals(-1, StringUtils.indexOfAnyBut("zzabyycdxx", (char[]) null));
assertEquals(-1, StringUtils.indexOfAnyBut("zzabyycdxx", new char[0]));
assertEquals(3, StringUtils.indexOfAnyBut("zzabyycdxx", new char[] {'z','a'}));
assertEquals(0, StringUtils.indexOfAnyBut("zzabyycdxx", new char[] {'b','y'}));
assertEquals(0, StringUtils.indexOfAnyBut("ab", new char[] {'z'}));
}
public void testIndexOfAnyBut_StringString() {
assertEquals(-1, StringUtils.indexOfAnyBut(null, (String) null));
assertEquals(-1, StringUtils.indexOfAnyBut(null, ""));
assertEquals(-1, StringUtils.indexOfAnyBut(null, "ab"));
assertEquals(-1, StringUtils.indexOfAnyBut("", (String) null));
assertEquals(-1, StringUtils.indexOfAnyBut("", ""));
assertEquals(-1, StringUtils.indexOfAnyBut("", "ab"));
assertEquals(-1, StringUtils.indexOfAnyBut("zzabyycdxx", (String) null));
assertEquals(-1, StringUtils.indexOfAnyBut("zzabyycdxx", ""));
assertEquals(3, StringUtils.indexOfAnyBut("zzabyycdxx", "za"));
assertEquals(0, StringUtils.indexOfAnyBut("zzabyycdxx", "by"));
assertEquals(0, StringUtils.indexOfAnyBut("ab", "z"));
}
//-----------------------------------------------------------------------
public void testContainsOnly_String() {
String str1 = "a";
String str2 = "b";
String str3 = "ab";
String chars1= "b";
String chars2= "a";
String chars3= "ab";
assertEquals(false, StringUtils.containsOnly(null, (String) null));
assertEquals(false, StringUtils.containsOnly("", (String) null));
assertEquals(false, StringUtils.containsOnly(null, ""));
assertEquals(false, StringUtils.containsOnly(str1, ""));
assertEquals(true, StringUtils.containsOnly("", ""));
assertEquals(true, StringUtils.containsOnly("", chars1));
assertEquals(false, StringUtils.containsOnly(str1, chars1));
assertEquals(true, StringUtils.containsOnly(str1, chars2));
assertEquals(true, StringUtils.containsOnly(str1, chars3));
assertEquals(true, StringUtils.containsOnly(str2, chars1));
assertEquals(false, StringUtils.containsOnly(str2, chars2));
assertEquals(true, StringUtils.containsOnly(str2, chars3));
assertEquals(false, StringUtils.containsOnly(str3, chars1));
assertEquals(false, StringUtils.containsOnly(str3, chars2));
assertEquals(true, StringUtils.containsOnly(str3, chars3));
}
public void testContainsOnly_Chararray() {
String str1 = "a";
String str2 = "b";
String str3 = "ab";
char[] chars1= {'b'};
char[] chars2= {'a'};
char[] chars3= {'a', 'b'};
char[] emptyChars = new char[0];
assertEquals(false, StringUtils.containsOnly(null, (char[]) null));
assertEquals(false, StringUtils.containsOnly("", (char[]) null));
assertEquals(false, StringUtils.containsOnly(null, emptyChars));
assertEquals(false, StringUtils.containsOnly(str1, emptyChars));
assertEquals(true, StringUtils.containsOnly("", emptyChars));
assertEquals(true, StringUtils.containsOnly("", chars1));
assertEquals(false, StringUtils.containsOnly(str1, chars1));
assertEquals(true, StringUtils.containsOnly(str1, chars2));
assertEquals(true, StringUtils.containsOnly(str1, chars3));
assertEquals(true, StringUtils.containsOnly(str2, chars1));
assertEquals(false, StringUtils.containsOnly(str2, chars2));
assertEquals(true, StringUtils.containsOnly(str2, chars3));
assertEquals(false, StringUtils.containsOnly(str3, chars1));
assertEquals(false, StringUtils.containsOnly(str3, chars2));
assertEquals(true, StringUtils.containsOnly(str3, chars3));
}
public void testContainsNone_String() {
String str1 = "a";
String str2 = "b";
String str3 = "ab.";
String chars1= "b";
String chars2= ".";
String chars3= "cd";
assertEquals(true, StringUtils.containsNone(null, (String) null));
assertEquals(true, StringUtils.containsNone("", (String) null));
assertEquals(true, StringUtils.containsNone(null, ""));
assertEquals(true, StringUtils.containsNone(str1, ""));
assertEquals(true, StringUtils.containsNone("", ""));
assertEquals(true, StringUtils.containsNone("", chars1));
assertEquals(true, StringUtils.containsNone(str1, chars1));
assertEquals(true, StringUtils.containsNone(str1, chars2));
assertEquals(true, StringUtils.containsNone(str1, chars3));
assertEquals(false, StringUtils.containsNone(str2, chars1));
assertEquals(true, StringUtils.containsNone(str2, chars2));
assertEquals(true, StringUtils.containsNone(str2, chars3));
assertEquals(false, StringUtils.containsNone(str3, chars1));
assertEquals(false, StringUtils.containsNone(str3, chars2));
assertEquals(true, StringUtils.containsNone(str3, chars3));
}
public void testContainsNone_Chararray() {
String str1 = "a";
String str2 = "b";
String str3 = "ab.";
char[] chars1= {'b'};
char[] chars2= {'.'};
char[] chars3= {'c', 'd'};
char[] emptyChars = new char[0];
assertEquals(true, StringUtils.containsNone(null, (char[]) null));
assertEquals(true, StringUtils.containsNone("", (char[]) null));
assertEquals(true, StringUtils.containsNone(null, emptyChars));
assertEquals(true, StringUtils.containsNone(str1, emptyChars));
assertEquals(true, StringUtils.containsNone("", emptyChars));
assertEquals(true, StringUtils.containsNone("", chars1));
assertEquals(true, StringUtils.containsNone(str1, chars1));
assertEquals(true, StringUtils.containsNone(str1, chars2));
assertEquals(true, StringUtils.containsNone(str1, chars3));
assertEquals(false, StringUtils.containsNone(str2, chars1));
assertEquals(true, StringUtils.containsNone(str2, chars2));
assertEquals(true, StringUtils.containsNone(str2, chars3));
assertEquals(false, StringUtils.containsNone(str3, chars1));
assertEquals(false, StringUtils.containsNone(str3, chars2));
assertEquals(true, StringUtils.containsNone(str3, chars3));
}
/**
* See http://java.sun.com/developer/technicalArticles/Intl/Supplementary/
*/
public void testContainsStringWithSupplementaryChars() {
assertEquals(true, StringUtils.contains(CharU20000 + CharU20001, CharU20000));
assertEquals(true, StringUtils.contains(CharU20000 + CharU20001, CharU20001));
assertEquals(true, StringUtils.contains(CharU20000, CharU20000));
assertEquals(false, StringUtils.contains(CharU20000, CharU20001));
}
/**
* See http://java.sun.com/developer/technicalArticles/Intl/Supplementary/
*/
public void testContainsAnyStringWithSupplementaryChars() {
assertEquals(true, StringUtils.containsAny(CharU20000 + CharU20001, CharU20000));
assertEquals(true, StringUtils.containsAny(CharU20000 + CharU20001, CharU20001));
assertEquals(true, StringUtils.containsAny(CharU20000, CharU20000));
// Sanity check:
assertEquals(-1, CharU20000.indexOf(CharU20001));
assertEquals(0, CharU20000.indexOf(CharU20001.charAt(0)));
assertEquals(-1, CharU20000.indexOf(CharU20001.charAt(1)));
// Test:
assertEquals(false, StringUtils.containsAny(CharU20000, CharU20001));
assertEquals(false, StringUtils.containsAny(CharU20001, CharU20000));
}
/**
* See http://java.sun.com/developer/technicalArticles/Intl/Supplementary/
*/
public void testContainsAnyCharArrayWithSupplementaryChars() {
assertEquals(true, StringUtils.containsAny(CharU20000 + CharU20001, CharU20000.toCharArray()));
assertEquals(true, StringUtils.containsAny(CharU20000 + CharU20001, CharU20001.toCharArray()));
assertEquals(true, StringUtils.containsAny(CharU20000, CharU20000.toCharArray()));
// Sanity check:
assertEquals(-1, CharU20000.indexOf(CharU20001));
assertEquals(0, CharU20000.indexOf(CharU20001.charAt(0)));
assertEquals(-1, CharU20000.indexOf(CharU20001.charAt(1)));
// Test:
assertEquals(false, StringUtils.containsAny(CharU20000, CharU20001.toCharArray()));
assertEquals(false, StringUtils.containsAny(CharU20001, CharU20000.toCharArray()));
}
} |
|
public void testInverseExternalId928() throws Exception
{
final String CLASS = Payload928.class.getName();
ObjectMapper mapper = new ObjectMapper();
final String successCase = "{\"payload\":{\"something\":\"test\"},\"class\":\""+CLASS+"\"}";
Envelope928 envelope1 = mapper.readValue(successCase, Envelope928.class);
assertNotNull(envelope1);
assertEquals(Payload928.class, envelope1._payload.getClass());
// and then re-ordered case that was problematic
final String failCase = "{\"class\":\""+CLASS+"\",\"payload\":{\"something\":\"test\"}}";
Envelope928 envelope2 = mapper.readValue(failCase, Envelope928.class);
assertNotNull(envelope2);
assertEquals(Payload928.class, envelope2._payload.getClass());
} | com.fasterxml.jackson.databind.jsontype.TestExternalId::testInverseExternalId928 | src/test/java/com/fasterxml/jackson/databind/jsontype/TestExternalId.java | 497 | src/test/java/com/fasterxml/jackson/databind/jsontype/TestExternalId.java | testInverseExternalId928 | package com.fasterxml.jackson.databind.jsontype;
import java.util.*;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.annotation.JsonTypeInfo.As;
import com.fasterxml.jackson.annotation.JsonTypeInfo.Id;
import com.fasterxml.jackson.databind.BaseMapTest;
import com.fasterxml.jackson.databind.ObjectMapper;
// Tests for External type id, one that exists at same level as typed Object,
// that is, property is not within typed object but a member of its parent.
public class TestExternalId extends BaseMapTest
{
static class ExternalBean
{
@JsonTypeInfo(use=Id.NAME, include=As.EXTERNAL_PROPERTY, property="extType")
public Object bean;
public ExternalBean() { }
public ExternalBean(int v) {
bean = new ValueBean(v);
}
}
// for [Issue#96]
static class ExternalBeanWithDefault
{
@JsonTypeInfo(use=Id.CLASS, include=As.EXTERNAL_PROPERTY, property="extType",
defaultImpl=ValueBean.class)
public Object bean;
public ExternalBeanWithDefault() { }
public ExternalBeanWithDefault(int v) {
bean = new ValueBean(v);
}
}
static class ExternalBean3
{
@JsonTypeInfo(use=Id.NAME, include=As.EXTERNAL_PROPERTY, property="extType1")
public Object value1;
@JsonTypeInfo(use=Id.NAME, include=As.EXTERNAL_PROPERTY, property="extType2")
public Object value2;
public int foo;
@JsonTypeInfo(use=Id.NAME, include=As.EXTERNAL_PROPERTY, property="extType3")
public Object value3;
public ExternalBean3() { }
public ExternalBean3(int v) {
value1 = new ValueBean(v);
value2 = new ValueBean(v+1);
value3 = new ValueBean(v+2);
foo = v;
}
}
static class ExternalBeanWithCreator
{
@JsonTypeInfo(use=Id.NAME, include=As.EXTERNAL_PROPERTY, property="extType")
public Object value;
public int foo;
@JsonCreator
public ExternalBeanWithCreator(@JsonProperty("foo") int f)
{
foo = f;
value = new ValueBean(f);
}
}
@JsonTypeName("vbean")
static class ValueBean {
public int value;
public ValueBean() { }
public ValueBean(int v) { value = v; }
}
@JsonTypeName("funk")
@JsonTypeInfo(use=Id.NAME, include=As.EXTERNAL_PROPERTY, property="extType")
static class FunkyExternalBean {
public int i = 3;
}
// [JACKSON-798]: problems with polymorphic types, external prop
@JsonSubTypes(value= { @JsonSubTypes.Type(value=Derived1.class, name="d1"),
@JsonSubTypes.Type(value=Derived2.class, name="d2") })
interface Base {
String getBaseProperty();
}
static class Derived1 implements Base {
private String derived1Property;
private String baseProperty;
protected Derived1() { throw new IllegalStateException("wrong constructor called"); }
@JsonCreator
public Derived1(@JsonProperty("derived1Property") String d1p,
@JsonProperty("baseProperty") String bp) {
derived1Property = d1p;
baseProperty = bp;
}
@Override
@JsonProperty public String getBaseProperty() {
return baseProperty;
}
@JsonProperty public String getDerived1Property() {
return derived1Property;
}
}
static class Derived2 implements Base {
private String derived2Property;
private String baseProperty;
protected Derived2() { throw new IllegalStateException("wrong constructor called"); }
@JsonCreator
public Derived2(@JsonProperty("derived2Property") String d2p,
@JsonProperty("baseProperty") String bp) {
derived2Property = d2p;
baseProperty = bp;
}
@Override
@JsonProperty public String getBaseProperty() {
return baseProperty;
}
@JsonProperty public String getDerived2Property() {
return derived2Property;
}
}
static class BaseContainer {
protected final Base base;
protected final String baseContainerProperty;
protected BaseContainer() { throw new IllegalStateException("wrong constructor called"); }
@JsonCreator
public BaseContainer(@JsonProperty("baseContainerProperty") String bcp, @JsonProperty("base") Base b) {
baseContainerProperty = bcp;
base = b;
}
@JsonProperty
public String getBaseContainerProperty() { return baseContainerProperty; }
@JsonTypeInfo(use=JsonTypeInfo.Id.NAME, include=JsonTypeInfo.As.EXTERNAL_PROPERTY, property="type")
@JsonProperty
public Base getBase() { return base; }
}
// [JACKSON-831]: should allow a property to map id to as well
interface Pet {}
static class Dog implements Pet {
public String name;
}
static class House831 {
protected String petType;
@JsonTypeInfo(use = Id.NAME, include = As.EXTERNAL_PROPERTY, property = "petType")
@JsonSubTypes({@JsonSubTypes.Type(name = "dog", value = Dog.class)})
public Pet pet;
public String getPetType() {
return petType;
}
public void setPetType(String petType) {
this.petType = petType;
}
}
// for [Issue#118]
static class ExternalTypeWithNonPOJO {
@JsonTypeInfo(use = JsonTypeInfo.Id.NAME,
property = "type",
visible = true,
include = JsonTypeInfo.As.EXTERNAL_PROPERTY,
defaultImpl = String.class)
@JsonSubTypes({
@JsonSubTypes.Type(value = Date.class, name = "date"),
@JsonSubTypes.Type(value = AsValueThingy.class, name = "thingy")
})
public Object value;
public ExternalTypeWithNonPOJO() { }
public ExternalTypeWithNonPOJO(Object o) { value = o; }
}
// for [Issue#119]
static class AsValueThingy {
public long rawDate;
public AsValueThingy(long l) { rawDate = l; }
public AsValueThingy() { }
@JsonValue public Date serialization() {
return new Date(rawDate);
}
}
// [databind#222]
static class Issue222Bean
{
@JsonTypeInfo(use = JsonTypeInfo.Id.NAME,
property = "type",
include = JsonTypeInfo.As.EXTERNAL_PROPERTY)
public Issue222BeanB value;
public String type = "foo";
public Issue222Bean() { }
public Issue222Bean(int v) {
value = new Issue222BeanB(v);
}
}
@JsonTypeName("222b") // shouldn't actually matter
static class Issue222BeanB
{
public int x;
public Issue222BeanB() { }
public Issue222BeanB(int value) { x = value; }
}
// [databind#928]
static class Envelope928 {
Object _payload;
public Envelope928(@JsonProperty("payload")
@JsonTypeInfo(use=JsonTypeInfo.Id.CLASS, include=JsonTypeInfo.As.EXTERNAL_PROPERTY, property="class")
Object payload) {
_payload = payload;
}
}
static class Payload928 {
public String something;
}
/*
/**********************************************************
/* Unit tests, serialization
/**********************************************************
*/
private final ObjectMapper MAPPER = new ObjectMapper();
public void testSimpleSerialization() throws Exception
{
ObjectMapper mapper = new ObjectMapper();
mapper.registerSubtypes(ValueBean.class);
// This may look odd, but one implementation nastiness is the fact
// that we can not properly serialize type id before the object,
// because call is made after property name (for object) has already
// been written out. So we'll write it after...
// Deserializer will work either way as it can not rely on ordering
// anyway.
assertEquals("{\"bean\":{\"value\":11},\"extType\":\"vbean\"}",
mapper.writeValueAsString(new ExternalBean(11)));
}
// If trying to use with Class, should just become "PROPERTY" instead:
public void testImproperExternalIdSerialization() throws Exception
{
ObjectMapper mapper = new ObjectMapper();
assertEquals("{\"extType\":\"funk\",\"i\":3}",
mapper.writeValueAsString(new FunkyExternalBean()));
}
/*
/**********************************************************
/* Unit tests, deserialization
/**********************************************************
*/
public void testSimpleDeserialization() throws Exception
{
ObjectMapper mapper = new ObjectMapper();
mapper.registerSubtypes(ValueBean.class);
ExternalBean result = mapper.readValue("{\"bean\":{\"value\":11},\"extType\":\"vbean\"}", ExternalBean.class);
assertNotNull(result);
assertNotNull(result.bean);
ValueBean vb = (ValueBean) result.bean;
assertEquals(11, vb.value);
// let's also test with order switched:
result = mapper.readValue("{\"extType\":\"vbean\", \"bean\":{\"value\":13}}", ExternalBean.class);
assertNotNull(result);
assertNotNull(result.bean);
vb = (ValueBean) result.bean;
assertEquals(13, vb.value);
}
// Test for verifying that it's ok to have multiple (say, 3)
// externally typed things, mixed with other stuff...
public void testMultipleTypeIdsDeserialization() throws Exception
{
ObjectMapper mapper = new ObjectMapper();
mapper.registerSubtypes(ValueBean.class);
String json = mapper.writeValueAsString(new ExternalBean3(3));
ExternalBean3 result = mapper.readValue(json, ExternalBean3.class);
assertNotNull(result);
assertNotNull(result.value1);
assertNotNull(result.value2);
assertNotNull(result.value3);
assertEquals(3, ((ValueBean)result.value1).value);
assertEquals(4, ((ValueBean)result.value2).value);
assertEquals(5, ((ValueBean)result.value3).value);
assertEquals(3, result.foo);
}
// Also, it should be ok to use @JsonCreator as well...
public void testExternalTypeWithCreator() throws Exception
{
ObjectMapper mapper = new ObjectMapper();
mapper.registerSubtypes(ValueBean.class);
String json = mapper.writeValueAsString(new ExternalBeanWithCreator(7));
ExternalBeanWithCreator result = mapper.readValue(json, ExternalBeanWithCreator.class);
assertNotNull(result);
assertNotNull(result.value);
assertEquals(7, ((ValueBean)result.value).value);
assertEquals(7, result.foo);
}
// If trying to use with Class, should just become "PROPERTY" instead:
public void testImproperExternalIdDeserialization() throws Exception
{
FunkyExternalBean result = MAPPER.readValue("{\"extType\":\"funk\",\"i\":3}",
FunkyExternalBean.class);
assertNotNull(result);
assertEquals(3, result.i);
result = MAPPER.readValue("{\"i\":4,\"extType\":\"funk\"}",
FunkyExternalBean.class);
assertNotNull(result);
assertEquals(4, result.i);
}
public void testIssue798() throws Exception
{
Base base = new Derived1("derived1 prop val", "base prop val");
BaseContainer baseContainer = new BaseContainer("bc prop val", base);
String generatedJson = MAPPER.writeValueAsString(baseContainer);
BaseContainer baseContainer2 = MAPPER.readValue(generatedJson,BaseContainer.class);
assertEquals("bc prop val", baseContainer.getBaseContainerProperty());
Base b = baseContainer2.getBase();
assertNotNull(b);
if (b.getClass() != Derived1.class) {
fail("Should have type Derived1, was "+b.getClass().getName());
}
Derived1 derived1 = (Derived1) b;
assertEquals("base prop val", derived1.getBaseProperty());
assertEquals("derived1 prop val", derived1.getDerived1Property());
}
// There seems to be some problems if type is also visible...
public void testIssue831() throws Exception
{
final String JSON = "{ \"petType\": \"dog\",\n"
+"\"pet\": { \"name\": \"Pluto\" }\n}";
House831 result = MAPPER.readValue(JSON, House831.class);
assertNotNull(result);
assertNotNull(result.pet);
assertSame(Dog.class, result.pet.getClass());
assertEquals("dog", result.petType);
}
// For [Issue#96]: should allow use of default impl, if property missing
/* 18-Jan-2013, tatu: Unfortunately this collides with [Issue#118], and I don't
* know what the best resolution is. For now at least
*/
/*
public void testWithDefaultAndMissing() throws Exception
{
ExternalBeanWithDefault input = new ExternalBeanWithDefault(13);
// baseline: include type, verify things work:
String fullJson = MAPPER.writeValueAsString(input);
ExternalBeanWithDefault output = MAPPER.readValue(fullJson, ExternalBeanWithDefault.class);
assertNotNull(output);
assertNotNull(output.bean);
// and then try without type info...
ExternalBeanWithDefault defaulted = MAPPER.readValue("{\"bean\":{\"value\":13}}",
ExternalBeanWithDefault.class);
assertNotNull(defaulted);
assertNotNull(defaulted.bean);
assertSame(ValueBean.class, defaulted.bean.getClass());
}
*/
// For [Issue#118]
// Note: String works fine, since no type id will used; other scalar types have issues
public void testWithScalar118() throws Exception
{
ExternalTypeWithNonPOJO input = new ExternalTypeWithNonPOJO(new java.util.Date(123L));
String json = MAPPER.writeValueAsString(input);
assertNotNull(json);
// and back just to be sure:
ExternalTypeWithNonPOJO result = MAPPER.readValue(json, ExternalTypeWithNonPOJO.class);
assertNotNull(result.value);
assertTrue(result.value instanceof java.util.Date);
}
// For [Issue#118] using "natural" type(s)
public void testWithNaturalScalar118() throws Exception
{
ExternalTypeWithNonPOJO input = new ExternalTypeWithNonPOJO(Integer.valueOf(13));
String json = MAPPER.writeValueAsString(input);
assertNotNull(json);
// and back just to be sure:
ExternalTypeWithNonPOJO result = MAPPER.readValue(json, ExternalTypeWithNonPOJO.class);
assertNotNull(result.value);
assertTrue(result.value instanceof Integer);
// ditto with others types
input = new ExternalTypeWithNonPOJO(Boolean.TRUE);
json = MAPPER.writeValueAsString(input);
assertNotNull(json);
result = MAPPER.readValue(json, ExternalTypeWithNonPOJO.class);
assertNotNull(result.value);
assertTrue(result.value instanceof Boolean);
input = new ExternalTypeWithNonPOJO("foobar");
json = MAPPER.writeValueAsString(input);
assertNotNull(json);
result = MAPPER.readValue(json, ExternalTypeWithNonPOJO.class);
assertNotNull(result.value);
assertTrue(result.value instanceof String);
assertEquals("foobar", result.value);
}
// For [databind#119]... and bit of [#167] as well
public void testWithAsValue() throws Exception
{
ExternalTypeWithNonPOJO input = new ExternalTypeWithNonPOJO(new AsValueThingy(12345L));
String json = MAPPER.writeValueAsString(input);
assertNotNull(json);
assertEquals("{\"value\":12345,\"type\":\"date\"}", json);
// and get it back too:
ExternalTypeWithNonPOJO result = MAPPER.readValue(json, ExternalTypeWithNonPOJO.class);
assertNotNull(result);
assertNotNull(result.value);
/* 13-Feb-2013, tatu: Urgh. I don't think this can work quite as intended...
* since POJO type, and type of thing @JsonValue annotated method returns
* are not related. Best we can do is thus this:
*/
/*
assertEquals(AsValueThingy.class, result.value.getClass());
assertEquals(12345L, ((AsValueThingy) result.value).rawDate);
*/
assertEquals(Date.class, result.value.getClass());
assertEquals(12345L, ((Date) result.value).getTime());
}
// for [databind#222]
public void testExternalTypeWithProp222() throws Exception
{
final ObjectMapper mapper = new ObjectMapper();
Issue222Bean input = new Issue222Bean(13);
String json = mapper.writeValueAsString(input);
assertEquals("{\"value\":{\"x\":13},\"type\":\"foo\"}", json);
}
// [databind#928]
public void testInverseExternalId928() throws Exception
{
final String CLASS = Payload928.class.getName();
ObjectMapper mapper = new ObjectMapper();
final String successCase = "{\"payload\":{\"something\":\"test\"},\"class\":\""+CLASS+"\"}";
Envelope928 envelope1 = mapper.readValue(successCase, Envelope928.class);
assertNotNull(envelope1);
assertEquals(Payload928.class, envelope1._payload.getClass());
// and then re-ordered case that was problematic
final String failCase = "{\"class\":\""+CLASS+"\",\"payload\":{\"something\":\"test\"}}";
Envelope928 envelope2 = mapper.readValue(failCase, Envelope928.class);
assertNotNull(envelope2);
assertEquals(Payload928.class, envelope2._payload.getClass());
}
} | // You are a professional Java test case writer, please create a test case named `testInverseExternalId928` for the issue `JacksonDatabind-928`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonDatabind-928
//
// ## Issue-Title:
// Problem deserializing External Type Id if type id comes before POJO
//
// ## Issue-Description:
// (note: seems to be similar or related to [FasterXML/jackson-module-afterburner#58](https://github.com/FasterXML/jackson-module-afterburner/issues/58))
//
//
// With 2.6, looks like handling of External Type Id is broken in some rare (?) cases; existing unit tests did not catch this. At this point I am speculating this is due to some refactoring, or change to use more efficient 'nextFieldName()' method.
//
//
//
//
public void testInverseExternalId928() throws Exception {
| 497 | // [databind#928] | 27 | 481 | src/test/java/com/fasterxml/jackson/databind/jsontype/TestExternalId.java | src/test/java | ```markdown
## Issue-ID: JacksonDatabind-928
## Issue-Title:
Problem deserializing External Type Id if type id comes before POJO
## Issue-Description:
(note: seems to be similar or related to [FasterXML/jackson-module-afterburner#58](https://github.com/FasterXML/jackson-module-afterburner/issues/58))
With 2.6, looks like handling of External Type Id is broken in some rare (?) cases; existing unit tests did not catch this. At this point I am speculating this is due to some refactoring, or change to use more efficient 'nextFieldName()' method.
```
You are a professional Java test case writer, please create a test case named `testInverseExternalId928` for the issue `JacksonDatabind-928`, utilizing the provided issue report information and the following function signature.
```java
public void testInverseExternalId928() throws Exception {
```
| 481 | [
"com.fasterxml.jackson.databind.deser.BeanDeserializer"
] | 7c1b1d64149ad3cbec36f0ea8a644e9cf4e659edd2962646bbd9531ac6ef1037 | public void testInverseExternalId928() throws Exception
| // You are a professional Java test case writer, please create a test case named `testInverseExternalId928` for the issue `JacksonDatabind-928`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonDatabind-928
//
// ## Issue-Title:
// Problem deserializing External Type Id if type id comes before POJO
//
// ## Issue-Description:
// (note: seems to be similar or related to [FasterXML/jackson-module-afterburner#58](https://github.com/FasterXML/jackson-module-afterburner/issues/58))
//
//
// With 2.6, looks like handling of External Type Id is broken in some rare (?) cases; existing unit tests did not catch this. At this point I am speculating this is due to some refactoring, or change to use more efficient 'nextFieldName()' method.
//
//
//
//
| JacksonDatabind | package com.fasterxml.jackson.databind.jsontype;
import java.util.*;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.annotation.JsonTypeInfo.As;
import com.fasterxml.jackson.annotation.JsonTypeInfo.Id;
import com.fasterxml.jackson.databind.BaseMapTest;
import com.fasterxml.jackson.databind.ObjectMapper;
// Tests for External type id, one that exists at same level as typed Object,
// that is, property is not within typed object but a member of its parent.
public class TestExternalId extends BaseMapTest
{
static class ExternalBean
{
@JsonTypeInfo(use=Id.NAME, include=As.EXTERNAL_PROPERTY, property="extType")
public Object bean;
public ExternalBean() { }
public ExternalBean(int v) {
bean = new ValueBean(v);
}
}
// for [Issue#96]
static class ExternalBeanWithDefault
{
@JsonTypeInfo(use=Id.CLASS, include=As.EXTERNAL_PROPERTY, property="extType",
defaultImpl=ValueBean.class)
public Object bean;
public ExternalBeanWithDefault() { }
public ExternalBeanWithDefault(int v) {
bean = new ValueBean(v);
}
}
static class ExternalBean3
{
@JsonTypeInfo(use=Id.NAME, include=As.EXTERNAL_PROPERTY, property="extType1")
public Object value1;
@JsonTypeInfo(use=Id.NAME, include=As.EXTERNAL_PROPERTY, property="extType2")
public Object value2;
public int foo;
@JsonTypeInfo(use=Id.NAME, include=As.EXTERNAL_PROPERTY, property="extType3")
public Object value3;
public ExternalBean3() { }
public ExternalBean3(int v) {
value1 = new ValueBean(v);
value2 = new ValueBean(v+1);
value3 = new ValueBean(v+2);
foo = v;
}
}
static class ExternalBeanWithCreator
{
@JsonTypeInfo(use=Id.NAME, include=As.EXTERNAL_PROPERTY, property="extType")
public Object value;
public int foo;
@JsonCreator
public ExternalBeanWithCreator(@JsonProperty("foo") int f)
{
foo = f;
value = new ValueBean(f);
}
}
@JsonTypeName("vbean")
static class ValueBean {
public int value;
public ValueBean() { }
public ValueBean(int v) { value = v; }
}
@JsonTypeName("funk")
@JsonTypeInfo(use=Id.NAME, include=As.EXTERNAL_PROPERTY, property="extType")
static class FunkyExternalBean {
public int i = 3;
}
// [JACKSON-798]: problems with polymorphic types, external prop
@JsonSubTypes(value= { @JsonSubTypes.Type(value=Derived1.class, name="d1"),
@JsonSubTypes.Type(value=Derived2.class, name="d2") })
interface Base {
String getBaseProperty();
}
static class Derived1 implements Base {
private String derived1Property;
private String baseProperty;
protected Derived1() { throw new IllegalStateException("wrong constructor called"); }
@JsonCreator
public Derived1(@JsonProperty("derived1Property") String d1p,
@JsonProperty("baseProperty") String bp) {
derived1Property = d1p;
baseProperty = bp;
}
@Override
@JsonProperty public String getBaseProperty() {
return baseProperty;
}
@JsonProperty public String getDerived1Property() {
return derived1Property;
}
}
static class Derived2 implements Base {
private String derived2Property;
private String baseProperty;
protected Derived2() { throw new IllegalStateException("wrong constructor called"); }
@JsonCreator
public Derived2(@JsonProperty("derived2Property") String d2p,
@JsonProperty("baseProperty") String bp) {
derived2Property = d2p;
baseProperty = bp;
}
@Override
@JsonProperty public String getBaseProperty() {
return baseProperty;
}
@JsonProperty public String getDerived2Property() {
return derived2Property;
}
}
static class BaseContainer {
protected final Base base;
protected final String baseContainerProperty;
protected BaseContainer() { throw new IllegalStateException("wrong constructor called"); }
@JsonCreator
public BaseContainer(@JsonProperty("baseContainerProperty") String bcp, @JsonProperty("base") Base b) {
baseContainerProperty = bcp;
base = b;
}
@JsonProperty
public String getBaseContainerProperty() { return baseContainerProperty; }
@JsonTypeInfo(use=JsonTypeInfo.Id.NAME, include=JsonTypeInfo.As.EXTERNAL_PROPERTY, property="type")
@JsonProperty
public Base getBase() { return base; }
}
// [JACKSON-831]: should allow a property to map id to as well
interface Pet {}
static class Dog implements Pet {
public String name;
}
static class House831 {
protected String petType;
@JsonTypeInfo(use = Id.NAME, include = As.EXTERNAL_PROPERTY, property = "petType")
@JsonSubTypes({@JsonSubTypes.Type(name = "dog", value = Dog.class)})
public Pet pet;
public String getPetType() {
return petType;
}
public void setPetType(String petType) {
this.petType = petType;
}
}
// for [Issue#118]
static class ExternalTypeWithNonPOJO {
@JsonTypeInfo(use = JsonTypeInfo.Id.NAME,
property = "type",
visible = true,
include = JsonTypeInfo.As.EXTERNAL_PROPERTY,
defaultImpl = String.class)
@JsonSubTypes({
@JsonSubTypes.Type(value = Date.class, name = "date"),
@JsonSubTypes.Type(value = AsValueThingy.class, name = "thingy")
})
public Object value;
public ExternalTypeWithNonPOJO() { }
public ExternalTypeWithNonPOJO(Object o) { value = o; }
}
// for [Issue#119]
static class AsValueThingy {
public long rawDate;
public AsValueThingy(long l) { rawDate = l; }
public AsValueThingy() { }
@JsonValue public Date serialization() {
return new Date(rawDate);
}
}
// [databind#222]
static class Issue222Bean
{
@JsonTypeInfo(use = JsonTypeInfo.Id.NAME,
property = "type",
include = JsonTypeInfo.As.EXTERNAL_PROPERTY)
public Issue222BeanB value;
public String type = "foo";
public Issue222Bean() { }
public Issue222Bean(int v) {
value = new Issue222BeanB(v);
}
}
@JsonTypeName("222b") // shouldn't actually matter
static class Issue222BeanB
{
public int x;
public Issue222BeanB() { }
public Issue222BeanB(int value) { x = value; }
}
// [databind#928]
static class Envelope928 {
Object _payload;
public Envelope928(@JsonProperty("payload")
@JsonTypeInfo(use=JsonTypeInfo.Id.CLASS, include=JsonTypeInfo.As.EXTERNAL_PROPERTY, property="class")
Object payload) {
_payload = payload;
}
}
static class Payload928 {
public String something;
}
/*
/**********************************************************
/* Unit tests, serialization
/**********************************************************
*/
private final ObjectMapper MAPPER = new ObjectMapper();
public void testSimpleSerialization() throws Exception
{
ObjectMapper mapper = new ObjectMapper();
mapper.registerSubtypes(ValueBean.class);
// This may look odd, but one implementation nastiness is the fact
// that we can not properly serialize type id before the object,
// because call is made after property name (for object) has already
// been written out. So we'll write it after...
// Deserializer will work either way as it can not rely on ordering
// anyway.
assertEquals("{\"bean\":{\"value\":11},\"extType\":\"vbean\"}",
mapper.writeValueAsString(new ExternalBean(11)));
}
// If trying to use with Class, should just become "PROPERTY" instead:
public void testImproperExternalIdSerialization() throws Exception
{
ObjectMapper mapper = new ObjectMapper();
assertEquals("{\"extType\":\"funk\",\"i\":3}",
mapper.writeValueAsString(new FunkyExternalBean()));
}
/*
/**********************************************************
/* Unit tests, deserialization
/**********************************************************
*/
public void testSimpleDeserialization() throws Exception
{
ObjectMapper mapper = new ObjectMapper();
mapper.registerSubtypes(ValueBean.class);
ExternalBean result = mapper.readValue("{\"bean\":{\"value\":11},\"extType\":\"vbean\"}", ExternalBean.class);
assertNotNull(result);
assertNotNull(result.bean);
ValueBean vb = (ValueBean) result.bean;
assertEquals(11, vb.value);
// let's also test with order switched:
result = mapper.readValue("{\"extType\":\"vbean\", \"bean\":{\"value\":13}}", ExternalBean.class);
assertNotNull(result);
assertNotNull(result.bean);
vb = (ValueBean) result.bean;
assertEquals(13, vb.value);
}
// Test for verifying that it's ok to have multiple (say, 3)
// externally typed things, mixed with other stuff...
public void testMultipleTypeIdsDeserialization() throws Exception
{
ObjectMapper mapper = new ObjectMapper();
mapper.registerSubtypes(ValueBean.class);
String json = mapper.writeValueAsString(new ExternalBean3(3));
ExternalBean3 result = mapper.readValue(json, ExternalBean3.class);
assertNotNull(result);
assertNotNull(result.value1);
assertNotNull(result.value2);
assertNotNull(result.value3);
assertEquals(3, ((ValueBean)result.value1).value);
assertEquals(4, ((ValueBean)result.value2).value);
assertEquals(5, ((ValueBean)result.value3).value);
assertEquals(3, result.foo);
}
// Also, it should be ok to use @JsonCreator as well...
public void testExternalTypeWithCreator() throws Exception
{
ObjectMapper mapper = new ObjectMapper();
mapper.registerSubtypes(ValueBean.class);
String json = mapper.writeValueAsString(new ExternalBeanWithCreator(7));
ExternalBeanWithCreator result = mapper.readValue(json, ExternalBeanWithCreator.class);
assertNotNull(result);
assertNotNull(result.value);
assertEquals(7, ((ValueBean)result.value).value);
assertEquals(7, result.foo);
}
// If trying to use with Class, should just become "PROPERTY" instead:
public void testImproperExternalIdDeserialization() throws Exception
{
FunkyExternalBean result = MAPPER.readValue("{\"extType\":\"funk\",\"i\":3}",
FunkyExternalBean.class);
assertNotNull(result);
assertEquals(3, result.i);
result = MAPPER.readValue("{\"i\":4,\"extType\":\"funk\"}",
FunkyExternalBean.class);
assertNotNull(result);
assertEquals(4, result.i);
}
public void testIssue798() throws Exception
{
Base base = new Derived1("derived1 prop val", "base prop val");
BaseContainer baseContainer = new BaseContainer("bc prop val", base);
String generatedJson = MAPPER.writeValueAsString(baseContainer);
BaseContainer baseContainer2 = MAPPER.readValue(generatedJson,BaseContainer.class);
assertEquals("bc prop val", baseContainer.getBaseContainerProperty());
Base b = baseContainer2.getBase();
assertNotNull(b);
if (b.getClass() != Derived1.class) {
fail("Should have type Derived1, was "+b.getClass().getName());
}
Derived1 derived1 = (Derived1) b;
assertEquals("base prop val", derived1.getBaseProperty());
assertEquals("derived1 prop val", derived1.getDerived1Property());
}
// There seems to be some problems if type is also visible...
public void testIssue831() throws Exception
{
final String JSON = "{ \"petType\": \"dog\",\n"
+"\"pet\": { \"name\": \"Pluto\" }\n}";
House831 result = MAPPER.readValue(JSON, House831.class);
assertNotNull(result);
assertNotNull(result.pet);
assertSame(Dog.class, result.pet.getClass());
assertEquals("dog", result.petType);
}
// For [Issue#96]: should allow use of default impl, if property missing
/* 18-Jan-2013, tatu: Unfortunately this collides with [Issue#118], and I don't
* know what the best resolution is. For now at least
*/
/*
public void testWithDefaultAndMissing() throws Exception
{
ExternalBeanWithDefault input = new ExternalBeanWithDefault(13);
// baseline: include type, verify things work:
String fullJson = MAPPER.writeValueAsString(input);
ExternalBeanWithDefault output = MAPPER.readValue(fullJson, ExternalBeanWithDefault.class);
assertNotNull(output);
assertNotNull(output.bean);
// and then try without type info...
ExternalBeanWithDefault defaulted = MAPPER.readValue("{\"bean\":{\"value\":13}}",
ExternalBeanWithDefault.class);
assertNotNull(defaulted);
assertNotNull(defaulted.bean);
assertSame(ValueBean.class, defaulted.bean.getClass());
}
*/
// For [Issue#118]
// Note: String works fine, since no type id will used; other scalar types have issues
public void testWithScalar118() throws Exception
{
ExternalTypeWithNonPOJO input = new ExternalTypeWithNonPOJO(new java.util.Date(123L));
String json = MAPPER.writeValueAsString(input);
assertNotNull(json);
// and back just to be sure:
ExternalTypeWithNonPOJO result = MAPPER.readValue(json, ExternalTypeWithNonPOJO.class);
assertNotNull(result.value);
assertTrue(result.value instanceof java.util.Date);
}
// For [Issue#118] using "natural" type(s)
public void testWithNaturalScalar118() throws Exception
{
ExternalTypeWithNonPOJO input = new ExternalTypeWithNonPOJO(Integer.valueOf(13));
String json = MAPPER.writeValueAsString(input);
assertNotNull(json);
// and back just to be sure:
ExternalTypeWithNonPOJO result = MAPPER.readValue(json, ExternalTypeWithNonPOJO.class);
assertNotNull(result.value);
assertTrue(result.value instanceof Integer);
// ditto with others types
input = new ExternalTypeWithNonPOJO(Boolean.TRUE);
json = MAPPER.writeValueAsString(input);
assertNotNull(json);
result = MAPPER.readValue(json, ExternalTypeWithNonPOJO.class);
assertNotNull(result.value);
assertTrue(result.value instanceof Boolean);
input = new ExternalTypeWithNonPOJO("foobar");
json = MAPPER.writeValueAsString(input);
assertNotNull(json);
result = MAPPER.readValue(json, ExternalTypeWithNonPOJO.class);
assertNotNull(result.value);
assertTrue(result.value instanceof String);
assertEquals("foobar", result.value);
}
// For [databind#119]... and bit of [#167] as well
public void testWithAsValue() throws Exception
{
ExternalTypeWithNonPOJO input = new ExternalTypeWithNonPOJO(new AsValueThingy(12345L));
String json = MAPPER.writeValueAsString(input);
assertNotNull(json);
assertEquals("{\"value\":12345,\"type\":\"date\"}", json);
// and get it back too:
ExternalTypeWithNonPOJO result = MAPPER.readValue(json, ExternalTypeWithNonPOJO.class);
assertNotNull(result);
assertNotNull(result.value);
/* 13-Feb-2013, tatu: Urgh. I don't think this can work quite as intended...
* since POJO type, and type of thing @JsonValue annotated method returns
* are not related. Best we can do is thus this:
*/
/*
assertEquals(AsValueThingy.class, result.value.getClass());
assertEquals(12345L, ((AsValueThingy) result.value).rawDate);
*/
assertEquals(Date.class, result.value.getClass());
assertEquals(12345L, ((Date) result.value).getTime());
}
// for [databind#222]
public void testExternalTypeWithProp222() throws Exception
{
final ObjectMapper mapper = new ObjectMapper();
Issue222Bean input = new Issue222Bean(13);
String json = mapper.writeValueAsString(input);
assertEquals("{\"value\":{\"x\":13},\"type\":\"foo\"}", json);
}
// [databind#928]
public void testInverseExternalId928() throws Exception
{
final String CLASS = Payload928.class.getName();
ObjectMapper mapper = new ObjectMapper();
final String successCase = "{\"payload\":{\"something\":\"test\"},\"class\":\""+CLASS+"\"}";
Envelope928 envelope1 = mapper.readValue(successCase, Envelope928.class);
assertNotNull(envelope1);
assertEquals(Payload928.class, envelope1._payload.getClass());
// and then re-ordered case that was problematic
final String failCase = "{\"class\":\""+CLASS+"\",\"payload\":{\"something\":\"test\"}}";
Envelope928 envelope2 = mapper.readValue(failCase, Envelope928.class);
assertNotNull(envelope2);
assertEquals(Payload928.class, envelope2._payload.getClass());
}
} |
|
public void testForOffsetHoursMinutes_int_int() {
assertEquals(DateTimeZone.UTC, DateTimeZone.forOffsetHoursMinutes(0, 0));
assertEquals(DateTimeZone.forID("+23:59"), DateTimeZone.forOffsetHoursMinutes(23, 59));
assertEquals(DateTimeZone.forID("+02:15"), DateTimeZone.forOffsetHoursMinutes(2, 15));
assertEquals(DateTimeZone.forID("+02:00"), DateTimeZone.forOffsetHoursMinutes(2, 0));
try {
DateTimeZone.forOffsetHoursMinutes(2, -15);
fail();
} catch (IllegalArgumentException ex) {}
assertEquals(DateTimeZone.forID("+00:15"), DateTimeZone.forOffsetHoursMinutes(0, 15));
assertEquals(DateTimeZone.forID("+00:00"), DateTimeZone.forOffsetHoursMinutes(0, 0));
assertEquals(DateTimeZone.forID("-00:15"), DateTimeZone.forOffsetHoursMinutes(0, -15));
assertEquals(DateTimeZone.forID("-02:00"), DateTimeZone.forOffsetHoursMinutes(-2, 0));
assertEquals(DateTimeZone.forID("-02:15"), DateTimeZone.forOffsetHoursMinutes(-2, -15));
assertEquals(DateTimeZone.forID("-02:15"), DateTimeZone.forOffsetHoursMinutes(-2, 15));
assertEquals(DateTimeZone.forID("-23:59"), DateTimeZone.forOffsetHoursMinutes(-23, 59));
try {
DateTimeZone.forOffsetHoursMinutes(2, 60);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.forOffsetHoursMinutes(-2, 60);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.forOffsetHoursMinutes(24, 0);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.forOffsetHoursMinutes(-24, 0);
fail();
} catch (IllegalArgumentException ex) {}
} | org.joda.time.TestDateTimeZone::testForOffsetHoursMinutes_int_int | src/test/java/org/joda/time/TestDateTimeZone.java | 338 | src/test/java/org/joda/time/TestDateTimeZone.java | testForOffsetHoursMinutes_int_int | /*
* Copyright 2001-2013 Stephen Colebourne
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.joda.time;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.io.PrintStream;
import java.lang.reflect.Modifier;
import java.security.AllPermission;
import java.security.CodeSource;
import java.security.Permission;
import java.security.PermissionCollection;
import java.security.Permissions;
import java.security.Policy;
import java.security.ProtectionDomain;
import java.text.DateFormatSymbols;
import java.util.HashSet;
import java.util.LinkedHashMap;
import java.util.Locale;
import java.util.Map;
import java.util.Set;
import java.util.TimeZone;
import junit.framework.TestCase;
import junit.framework.TestSuite;
import org.joda.time.tz.DefaultNameProvider;
import org.joda.time.tz.NameProvider;
import org.joda.time.tz.Provider;
import org.joda.time.tz.UTCProvider;
import org.joda.time.tz.ZoneInfoProvider;
/**
* This class is a JUnit test for DateTimeZone.
*
* @author Stephen Colebourne
*/
public class TestDateTimeZone extends TestCase {
private static final boolean OLD_JDK;
static {
String str = System.getProperty("java.version");
boolean old = true;
if (str.length() > 3 &&
str.charAt(0) == '1' &&
str.charAt(1) == '.' &&
(str.charAt(2) == '4' || str.charAt(2) == '5' || str.charAt(2) == '6')) {
old = false;
}
OLD_JDK = old;
}
// Test in 2002/03 as time zones are more well known
// (before the late 90's they were all over the place)
private static final DateTimeZone PARIS = DateTimeZone.forID("Europe/Paris");
private static final DateTimeZone LONDON = DateTimeZone.forID("Europe/London");
long y2002days = 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 +
365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365;
long y2003days = 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 +
365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365 + 365;
// 2002-06-09
private long TEST_TIME_SUMMER =
(y2002days + 31L + 28L + 31L + 30L + 31L + 9L -1L) * DateTimeConstants.MILLIS_PER_DAY;
// 2002-01-09
private long TEST_TIME_WINTER =
(y2002days + 9L -1L) * DateTimeConstants.MILLIS_PER_DAY;
// // 2002-04-05 Fri
// private long TEST_TIME1 =
// (y2002days + 31L + 28L + 31L + 5L -1L) * DateTimeConstants.MILLIS_PER_DAY
// + 12L * DateTimeConstants.MILLIS_PER_HOUR
// + 24L * DateTimeConstants.MILLIS_PER_MINUTE;
//
// // 2003-05-06 Tue
// private long TEST_TIME2 =
// (y2003days + 31L + 28L + 31L + 30L + 6L -1L) * DateTimeConstants.MILLIS_PER_DAY
// + 14L * DateTimeConstants.MILLIS_PER_HOUR
// + 28L * DateTimeConstants.MILLIS_PER_MINUTE;
private static final Policy RESTRICT;
private static final Policy ALLOW;
static {
// don't call Policy.getPolicy()
RESTRICT = new Policy() {
public PermissionCollection getPermissions(CodeSource codesource) {
Permissions p = new Permissions();
p.add(new AllPermission()); // enable everything
return p;
}
public void refresh() {
}
public boolean implies(ProtectionDomain domain, Permission permission) {
if (permission instanceof JodaTimePermission) {
return false;
}
return true;
// return super.implies(domain, permission);
}
};
ALLOW = new Policy() {
public PermissionCollection getPermissions(CodeSource codesource) {
Permissions p = new Permissions();
p.add(new AllPermission()); // enable everything
return p;
}
public void refresh() {
}
};
}
private DateTimeZone zone;
private Locale locale;
public static void main(String[] args) {
junit.textui.TestRunner.run(suite());
}
public static TestSuite suite() {
return new TestSuite(TestDateTimeZone.class);
}
public TestDateTimeZone(String name) {
super(name);
}
protected void setUp() throws Exception {
locale = Locale.getDefault();
zone = DateTimeZone.getDefault();
Locale.setDefault(Locale.UK);
}
protected void tearDown() throws Exception {
Locale.setDefault(locale);
DateTimeZone.setDefault(zone);
}
//-----------------------------------------------------------------------
public void testDefault() {
assertNotNull(DateTimeZone.getDefault());
DateTimeZone.setDefault(PARIS);
assertSame(PARIS, DateTimeZone.getDefault());
try {
DateTimeZone.setDefault(null);
fail();
} catch (IllegalArgumentException ex) {}
}
public void testDefaultSecurity() {
if (OLD_JDK) {
return;
}
try {
Policy.setPolicy(RESTRICT);
System.setSecurityManager(new SecurityManager());
DateTimeZone.setDefault(PARIS);
fail();
} catch (SecurityException ex) {
// ok
} finally {
System.setSecurityManager(null);
Policy.setPolicy(ALLOW);
}
}
//-----------------------------------------------------------------------
public void testForID_String() {
assertEquals(DateTimeZone.getDefault(), DateTimeZone.forID((String) null));
DateTimeZone zone = DateTimeZone.forID("Europe/London");
assertEquals("Europe/London", zone.getID());
zone = DateTimeZone.forID("UTC");
assertSame(DateTimeZone.UTC, zone);
zone = DateTimeZone.forID("+00:00");
assertSame(DateTimeZone.UTC, zone);
zone = DateTimeZone.forID("+00");
assertSame(DateTimeZone.UTC, zone);
zone = DateTimeZone.forID("+01:23");
assertEquals("+01:23", zone.getID());
assertEquals(DateTimeConstants.MILLIS_PER_HOUR + (23L * DateTimeConstants.MILLIS_PER_MINUTE),
zone.getOffset(TEST_TIME_SUMMER));
zone = DateTimeZone.forID("-02:00");
assertEquals("-02:00", zone.getID());
assertEquals((-2L * DateTimeConstants.MILLIS_PER_HOUR),
zone.getOffset(TEST_TIME_SUMMER));
zone = DateTimeZone.forID("-07:05:34.0");
assertEquals("-07:05:34", zone.getID());
assertEquals((-7L * DateTimeConstants.MILLIS_PER_HOUR) +
(-5L * DateTimeConstants.MILLIS_PER_MINUTE) +
(-34L * DateTimeConstants.MILLIS_PER_SECOND),
zone.getOffset(TEST_TIME_SUMMER));
try {
DateTimeZone.forID("SST");
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.forID("europe/london");
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.forID("Europe/UK");
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.forID("+");
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.forID("+0");
fail();
} catch (IllegalArgumentException ex) {}
}
public void testForID_String_old() {
Map<String, String> map = new LinkedHashMap<String, String>();
map.put("GMT", "UTC");
map.put("WET", "WET");
map.put("CET", "CET");
map.put("MET", "CET");
map.put("ECT", "CET");
map.put("EET", "EET");
map.put("MIT", "Pacific/Apia");
map.put("HST", "Pacific/Honolulu");
map.put("AST", "America/Anchorage");
map.put("PST", "America/Los_Angeles");
map.put("MST", "America/Denver");
map.put("PNT", "America/Phoenix");
map.put("CST", "America/Chicago");
map.put("EST", "America/New_York");
map.put("IET", "America/Indiana/Indianapolis");
map.put("PRT", "America/Puerto_Rico");
map.put("CNT", "America/St_Johns");
map.put("AGT", "America/Argentina/Buenos_Aires");
map.put("BET", "America/Sao_Paulo");
map.put("ART", "Africa/Cairo");
map.put("CAT", "Africa/Harare");
map.put("EAT", "Africa/Addis_Ababa");
map.put("NET", "Asia/Yerevan");
map.put("PLT", "Asia/Karachi");
map.put("IST", "Asia/Kolkata");
map.put("BST", "Asia/Dhaka");
map.put("VST", "Asia/Ho_Chi_Minh");
map.put("CTT", "Asia/Shanghai");
map.put("JST", "Asia/Tokyo");
map.put("ACT", "Australia/Darwin");
map.put("AET", "Australia/Sydney");
map.put("SST", "Pacific/Guadalcanal");
map.put("NST", "Pacific/Auckland");
for (String key : map.keySet()) {
String value = map.get(key);
TimeZone juZone = TimeZone.getTimeZone(key);
DateTimeZone zone = DateTimeZone.forTimeZone(juZone);
assertEquals(value, zone.getID());
// System.out.println(juZone);
// System.out.println(juZone.getDisplayName());
// System.out.println(zone);
// System.out.println("------");
}
}
//-----------------------------------------------------------------------
public void testForOffsetHours_int() {
assertEquals(DateTimeZone.UTC, DateTimeZone.forOffsetHours(0));
assertEquals(DateTimeZone.forID("+03:00"), DateTimeZone.forOffsetHours(3));
assertEquals(DateTimeZone.forID("-02:00"), DateTimeZone.forOffsetHours(-2));
try {
DateTimeZone.forOffsetHours(999999);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testForOffsetHoursMinutes_int_int() {
assertEquals(DateTimeZone.UTC, DateTimeZone.forOffsetHoursMinutes(0, 0));
assertEquals(DateTimeZone.forID("+23:59"), DateTimeZone.forOffsetHoursMinutes(23, 59));
assertEquals(DateTimeZone.forID("+02:15"), DateTimeZone.forOffsetHoursMinutes(2, 15));
assertEquals(DateTimeZone.forID("+02:00"), DateTimeZone.forOffsetHoursMinutes(2, 0));
try {
DateTimeZone.forOffsetHoursMinutes(2, -15);
fail();
} catch (IllegalArgumentException ex) {}
assertEquals(DateTimeZone.forID("+00:15"), DateTimeZone.forOffsetHoursMinutes(0, 15));
assertEquals(DateTimeZone.forID("+00:00"), DateTimeZone.forOffsetHoursMinutes(0, 0));
assertEquals(DateTimeZone.forID("-00:15"), DateTimeZone.forOffsetHoursMinutes(0, -15));
assertEquals(DateTimeZone.forID("-02:00"), DateTimeZone.forOffsetHoursMinutes(-2, 0));
assertEquals(DateTimeZone.forID("-02:15"), DateTimeZone.forOffsetHoursMinutes(-2, -15));
assertEquals(DateTimeZone.forID("-02:15"), DateTimeZone.forOffsetHoursMinutes(-2, 15));
assertEquals(DateTimeZone.forID("-23:59"), DateTimeZone.forOffsetHoursMinutes(-23, 59));
try {
DateTimeZone.forOffsetHoursMinutes(2, 60);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.forOffsetHoursMinutes(-2, 60);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.forOffsetHoursMinutes(24, 0);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.forOffsetHoursMinutes(-24, 0);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testForOffsetMillis_int() {
assertSame(DateTimeZone.UTC, DateTimeZone.forOffsetMillis(0));
assertEquals(DateTimeZone.forID("+23:59:59.999"), DateTimeZone.forOffsetMillis((24 * 60 * 60 * 1000) - 1));
assertEquals(DateTimeZone.forID("+03:00"), DateTimeZone.forOffsetMillis(3 * 60 * 60 * 1000));
assertEquals(DateTimeZone.forID("-02:00"), DateTimeZone.forOffsetMillis(-2 * 60 * 60 * 1000));
assertEquals(DateTimeZone.forID("-23:59:59.999"), DateTimeZone.forOffsetMillis((-24 * 60 * 60 * 1000) + 1));
assertEquals(DateTimeZone.forID("+04:45:17.045"),
DateTimeZone.forOffsetMillis(
4 * 60 * 60 * 1000 + 45 * 60 * 1000 + 17 * 1000 + 45));
}
//-----------------------------------------------------------------------
public void testForTimeZone_TimeZone() {
assertEquals(DateTimeZone.getDefault(), DateTimeZone.forTimeZone((TimeZone) null));
DateTimeZone zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("Europe/London"));
assertEquals("Europe/London", zone.getID());
assertSame(DateTimeZone.UTC, DateTimeZone.forTimeZone(TimeZone.getTimeZone("UTC")));
zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("+00:00"));
assertSame(DateTimeZone.UTC, zone);
zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("GMT+00:00"));
assertSame(DateTimeZone.UTC, zone);
zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("GMT+00:00"));
assertSame(DateTimeZone.UTC, zone);
zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("GMT+00"));
assertSame(DateTimeZone.UTC, zone);
zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("GMT+01:23"));
assertEquals("+01:23", zone.getID());
assertEquals(DateTimeConstants.MILLIS_PER_HOUR + (23L * DateTimeConstants.MILLIS_PER_MINUTE),
zone.getOffset(TEST_TIME_SUMMER));
zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("GMT+1:23"));
assertEquals("+01:23", zone.getID());
assertEquals(DateTimeConstants.MILLIS_PER_HOUR + (23L * DateTimeConstants.MILLIS_PER_MINUTE),
zone.getOffset(TEST_TIME_SUMMER));
zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("GMT-02:00"));
assertEquals("-02:00", zone.getID());
assertEquals((-2L * DateTimeConstants.MILLIS_PER_HOUR), zone.getOffset(TEST_TIME_SUMMER));
zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("GMT+2"));
assertEquals("+02:00", zone.getID());
assertEquals((2L * DateTimeConstants.MILLIS_PER_HOUR), zone.getOffset(TEST_TIME_SUMMER));
zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("EST"));
assertEquals("America/New_York", zone.getID());
}
public void testTimeZoneConversion() {
TimeZone jdkTimeZone = TimeZone.getTimeZone("GMT-10");
assertEquals("GMT-10:00", jdkTimeZone.getID());
DateTimeZone jodaTimeZone = DateTimeZone.forTimeZone(jdkTimeZone);
assertEquals("-10:00", jodaTimeZone.getID());
assertEquals(jdkTimeZone.getRawOffset(), jodaTimeZone.getOffset(0L));
TimeZone convertedTimeZone = jodaTimeZone.toTimeZone();
assertEquals("GMT-10:00", jdkTimeZone.getID());
assertEquals(jdkTimeZone.getID(), convertedTimeZone.getID());
assertEquals(jdkTimeZone.getRawOffset(), convertedTimeZone.getRawOffset());
}
//-----------------------------------------------------------------------
public void testGetAvailableIDs() {
assertTrue(DateTimeZone.getAvailableIDs().contains("UTC"));
}
//-----------------------------------------------------------------------
public void testProvider() {
try {
assertNotNull(DateTimeZone.getProvider());
Provider provider = DateTimeZone.getProvider();
DateTimeZone.setProvider(null);
assertEquals(provider.getClass(), DateTimeZone.getProvider().getClass());
try {
DateTimeZone.setProvider(new MockNullIDSProvider());
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.setProvider(new MockEmptyIDSProvider());
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.setProvider(new MockNoUTCProvider());
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.setProvider(new MockBadUTCProvider());
fail();
} catch (IllegalArgumentException ex) {}
Provider prov = new MockOKProvider();
DateTimeZone.setProvider(prov);
assertSame(prov, DateTimeZone.getProvider());
assertEquals(2, DateTimeZone.getAvailableIDs().size());
assertTrue(DateTimeZone.getAvailableIDs().contains("UTC"));
assertTrue(DateTimeZone.getAvailableIDs().contains("Europe/London"));
} finally {
DateTimeZone.setProvider(null);
assertEquals(ZoneInfoProvider.class, DateTimeZone.getProvider().getClass());
}
try {
System.setProperty("org.joda.time.DateTimeZone.Provider", "org.joda.time.tz.UTCProvider");
DateTimeZone.setProvider(null);
assertEquals(UTCProvider.class, DateTimeZone.getProvider().getClass());
} finally {
System.getProperties().remove("org.joda.time.DateTimeZone.Provider");
DateTimeZone.setProvider(null);
assertEquals(ZoneInfoProvider.class, DateTimeZone.getProvider().getClass());
}
PrintStream syserr = System.err;
try {
System.setProperty("org.joda.time.DateTimeZone.Provider", "xxx");
ByteArrayOutputStream baos = new ByteArrayOutputStream();
System.setErr(new PrintStream(baos));
DateTimeZone.setProvider(null);
assertEquals(ZoneInfoProvider.class, DateTimeZone.getProvider().getClass());
String str = new String(baos.toByteArray());
assertTrue(str.indexOf("java.lang.ClassNotFoundException") >= 0);
} finally {
System.setErr(syserr);
System.getProperties().remove("org.joda.time.DateTimeZone.Provider");
DateTimeZone.setProvider(null);
assertEquals(ZoneInfoProvider.class, DateTimeZone.getProvider().getClass());
}
}
public void testProviderSecurity() {
if (OLD_JDK) {
return;
}
try {
Policy.setPolicy(RESTRICT);
System.setSecurityManager(new SecurityManager());
DateTimeZone.setProvider(new MockOKProvider());
fail();
} catch (SecurityException ex) {
// ok
} finally {
System.setSecurityManager(null);
Policy.setPolicy(ALLOW);
}
}
static class MockNullIDSProvider implements Provider {
public Set getAvailableIDs() {
return null;
}
public DateTimeZone getZone(String id) {
return null;
}
}
static class MockEmptyIDSProvider implements Provider {
public Set getAvailableIDs() {
return new HashSet();
}
public DateTimeZone getZone(String id) {
return null;
}
}
static class MockNoUTCProvider implements Provider {
public Set getAvailableIDs() {
Set set = new HashSet();
set.add("Europe/London");
return set;
}
public DateTimeZone getZone(String id) {
return null;
}
}
static class MockBadUTCProvider implements Provider {
public Set getAvailableIDs() {
Set set = new HashSet();
set.add("UTC");
set.add("Europe/London");
return set;
}
public DateTimeZone getZone(String id) {
return null;
}
}
static class MockOKProvider implements Provider {
public Set getAvailableIDs() {
Set set = new HashSet();
set.add("UTC");
set.add("Europe/London");
return set;
}
public DateTimeZone getZone(String id) {
return DateTimeZone.UTC;
}
}
//-----------------------------------------------------------------------
public void testNameProvider() {
try {
assertNotNull(DateTimeZone.getNameProvider());
NameProvider provider = DateTimeZone.getNameProvider();
DateTimeZone.setNameProvider(null);
assertEquals(provider.getClass(), DateTimeZone.getNameProvider().getClass());
provider = new MockOKButNullNameProvider();
DateTimeZone.setNameProvider(provider);
assertSame(provider, DateTimeZone.getNameProvider());
assertEquals("+00:00", DateTimeZone.UTC.getShortName(TEST_TIME_SUMMER));
assertEquals("+00:00", DateTimeZone.UTC.getName(TEST_TIME_SUMMER));
} finally {
DateTimeZone.setNameProvider(null);
}
try {
System.setProperty("org.joda.time.DateTimeZone.NameProvider", "org.joda.time.tz.DefaultNameProvider");
DateTimeZone.setNameProvider(null);
assertEquals(DefaultNameProvider.class, DateTimeZone.getNameProvider().getClass());
} finally {
System.getProperties().remove("org.joda.time.DateTimeZone.NameProvider");
DateTimeZone.setNameProvider(null);
assertEquals(DefaultNameProvider.class, DateTimeZone.getNameProvider().getClass());
}
PrintStream syserr = System.err;
try {
System.setProperty("org.joda.time.DateTimeZone.NameProvider", "xxx");
ByteArrayOutputStream baos = new ByteArrayOutputStream();
System.setErr(new PrintStream(baos));
DateTimeZone.setNameProvider(null);
assertEquals(DefaultNameProvider.class, DateTimeZone.getNameProvider().getClass());
String str = new String(baos.toByteArray());
assertTrue(str.indexOf("java.lang.ClassNotFoundException") >= 0);
} finally {
System.setErr(syserr);
System.getProperties().remove("org.joda.time.DateTimeZone.NameProvider");
DateTimeZone.setNameProvider(null);
assertEquals(DefaultNameProvider.class, DateTimeZone.getNameProvider().getClass());
}
}
public void testNameProviderSecurity() {
if (OLD_JDK) {
return;
}
try {
Policy.setPolicy(RESTRICT);
System.setSecurityManager(new SecurityManager());
DateTimeZone.setNameProvider(new MockOKButNullNameProvider());
fail();
} catch (SecurityException ex) {
// ok
} finally {
System.setSecurityManager(null);
Policy.setPolicy(ALLOW);
}
}
static class MockOKButNullNameProvider implements NameProvider {
public String getShortName(Locale locale, String id, String nameKey) {
return null;
}
public String getName(Locale locale, String id, String nameKey) {
return null;
}
}
//-----------------------------------------------------------------------
public void testConstructor() {
assertEquals(1, DateTimeZone.class.getDeclaredConstructors().length);
assertTrue(Modifier.isProtected(DateTimeZone.class.getDeclaredConstructors()[0].getModifiers()));
try {
new DateTimeZone(null) {
public String getNameKey(long instant) {
return null;
}
public int getOffset(long instant) {
return 0;
}
public int getStandardOffset(long instant) {
return 0;
}
public boolean isFixed() {
return false;
}
public long nextTransition(long instant) {
return 0;
}
public long previousTransition(long instant) {
return 0;
}
public boolean equals(Object object) {
return false;
}
};
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testGetID() {
DateTimeZone zone = DateTimeZone.forID("Europe/Paris");
assertEquals("Europe/Paris", zone.getID());
}
public void testGetNameKey() {
DateTimeZone zone = DateTimeZone.forID("Europe/London");
assertEquals("BST", zone.getNameKey(TEST_TIME_SUMMER));
assertEquals("GMT", zone.getNameKey(TEST_TIME_WINTER));
}
static final boolean JDK6;
static {
boolean jdk6 = true;
try {
DateFormatSymbols.class.getMethod("getInstance", new Class[] {Locale.class});
} catch (Exception ex) {
jdk6 = false;
}
JDK6 = jdk6;
}
public void testGetShortName() {}
// Defects4J: flaky method
// public void testGetShortName() {
// DateTimeZone zone = DateTimeZone.forID("Europe/London");
// assertEquals("BST", zone.getShortName(TEST_TIME_SUMMER));
// assertEquals("GMT", zone.getShortName(TEST_TIME_WINTER));
// assertEquals("BST", zone.getShortName(TEST_TIME_SUMMER, Locale.ENGLISH));
// }
public void testGetShortName_berlin() {}
// Defects4J: flaky method
// public void testGetShortName_berlin() {
// DateTimeZone berlin = DateTimeZone.forID("Europe/Berlin");
// assertEquals("CET", berlin.getShortName(TEST_TIME_WINTER, Locale.ENGLISH));
// assertEquals("CEST", berlin.getShortName(TEST_TIME_SUMMER, Locale.ENGLISH));
// if (JDK6) {
// assertEquals("MEZ", berlin.getShortName(TEST_TIME_WINTER, Locale.GERMAN));
// assertEquals("MESZ", berlin.getShortName(TEST_TIME_SUMMER, Locale.GERMAN));
// } else {
// assertEquals("CET", berlin.getShortName(TEST_TIME_WINTER, Locale.GERMAN));
// assertEquals("CEST", berlin.getShortName(TEST_TIME_SUMMER, Locale.GERMAN));
// }
// }
public void testGetShortNameProviderName() {
assertEquals(null, DateTimeZone.getNameProvider().getShortName(null, "Europe/London", "BST"));
assertEquals(null, DateTimeZone.getNameProvider().getShortName(Locale.ENGLISH, null, "BST"));
assertEquals(null, DateTimeZone.getNameProvider().getShortName(Locale.ENGLISH, "Europe/London", null));
assertEquals(null, DateTimeZone.getNameProvider().getShortName(null, null, null));
}
public void testGetShortNameNullKey() {
DateTimeZone zone = new MockDateTimeZone("Europe/London");
assertEquals("Europe/London", zone.getShortName(TEST_TIME_SUMMER, Locale.ENGLISH));
}
public void testGetName() {}
// Defects4J: flaky method
// public void testGetName() {
// DateTimeZone zone = DateTimeZone.forID("Europe/London");
// assertEquals("British Summer Time", zone.getName(TEST_TIME_SUMMER));
// assertEquals("Greenwich Mean Time", zone.getName(TEST_TIME_WINTER));
// assertEquals("British Summer Time", zone.getName(TEST_TIME_SUMMER, Locale.ENGLISH));
// }
public void testGetName_berlin() {}
// Defects4J: flaky method
// public void testGetName_berlin() {
// DateTimeZone berlin = DateTimeZone.forID("Europe/Berlin");
// assertEquals("Central European Time", berlin.getName(TEST_TIME_WINTER, Locale.ENGLISH));
// assertEquals("Central European Summer Time", berlin.getName(TEST_TIME_SUMMER, Locale.ENGLISH));
// if (JDK6) {
// assertEquals("Mitteleurop\u00e4ische Zeit", berlin.getName(TEST_TIME_WINTER, Locale.GERMAN));
// assertEquals("Mitteleurop\u00e4ische Sommerzeit", berlin.getName(TEST_TIME_SUMMER, Locale.GERMAN));
// } else {
// assertEquals("Zentraleurop\u00e4ische Zeit", berlin.getName(TEST_TIME_WINTER, Locale.GERMAN));
// assertEquals("Zentraleurop\u00e4ische Sommerzeit", berlin.getName(TEST_TIME_SUMMER, Locale.GERMAN));
// }
// }
public void testGetNameProviderName() {
assertEquals(null, DateTimeZone.getNameProvider().getName(null, "Europe/London", "BST"));
assertEquals(null, DateTimeZone.getNameProvider().getName(Locale.ENGLISH, null, "BST"));
assertEquals(null, DateTimeZone.getNameProvider().getName(Locale.ENGLISH, "Europe/London", null));
assertEquals(null, DateTimeZone.getNameProvider().getName(null, null, null));
}
public void testGetNameNullKey() {
DateTimeZone zone = new MockDateTimeZone("Europe/London");
assertEquals("Europe/London", zone.getName(TEST_TIME_SUMMER, Locale.ENGLISH));
}
static class MockDateTimeZone extends DateTimeZone {
public MockDateTimeZone(String id) {
super(id);
}
public String getNameKey(long instant) {
return null; // null
}
public int getOffset(long instant) {
return 0;
}
public int getStandardOffset(long instant) {
return 0;
}
public boolean isFixed() {
return false;
}
public long nextTransition(long instant) {
return 0;
}
public long previousTransition(long instant) {
return 0;
}
public boolean equals(Object object) {
return false;
}
}
//-----------------------------------------------------------------------
public void testGetOffset_long() {
DateTimeZone zone = DateTimeZone.forID("Europe/Paris");
assertEquals(2L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffset(TEST_TIME_SUMMER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffset(TEST_TIME_WINTER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getStandardOffset(TEST_TIME_SUMMER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getStandardOffset(TEST_TIME_WINTER));
assertEquals(2L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffsetFromLocal(TEST_TIME_SUMMER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffsetFromLocal(TEST_TIME_WINTER));
assertEquals(false, zone.isStandardOffset(TEST_TIME_SUMMER));
assertEquals(true, zone.isStandardOffset(TEST_TIME_WINTER));
}
public void testGetOffset_RI() {
DateTimeZone zone = DateTimeZone.forID("Europe/Paris");
assertEquals(2L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffset(new Instant(TEST_TIME_SUMMER)));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffset(new Instant(TEST_TIME_WINTER)));
assertEquals(zone.getOffset(DateTimeUtils.currentTimeMillis()), zone.getOffset(null));
}
public void testGetOffsetFixed() {
DateTimeZone zone = DateTimeZone.forID("+01:00");
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffset(TEST_TIME_SUMMER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffset(TEST_TIME_WINTER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getStandardOffset(TEST_TIME_SUMMER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getStandardOffset(TEST_TIME_WINTER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffsetFromLocal(TEST_TIME_SUMMER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffsetFromLocal(TEST_TIME_WINTER));
assertEquals(true, zone.isStandardOffset(TEST_TIME_SUMMER));
assertEquals(true, zone.isStandardOffset(TEST_TIME_WINTER));
}
public void testGetOffsetFixed_RI() {
DateTimeZone zone = DateTimeZone.forID("+01:00");
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffset(new Instant(TEST_TIME_SUMMER)));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffset(new Instant(TEST_TIME_WINTER)));
assertEquals(zone.getOffset(DateTimeUtils.currentTimeMillis()), zone.getOffset(null));
}
//-----------------------------------------------------------------------
public void testGetMillisKeepLocal() {
long millisLondon = TEST_TIME_SUMMER;
long millisParis = TEST_TIME_SUMMER - 1L * DateTimeConstants.MILLIS_PER_HOUR;
assertEquals(millisLondon, LONDON.getMillisKeepLocal(LONDON, millisLondon));
assertEquals(millisParis, LONDON.getMillisKeepLocal(LONDON, millisParis));
assertEquals(millisLondon, PARIS.getMillisKeepLocal(PARIS, millisLondon));
assertEquals(millisParis, PARIS.getMillisKeepLocal(PARIS, millisParis));
assertEquals(millisParis, LONDON.getMillisKeepLocal(PARIS, millisLondon));
assertEquals(millisLondon, PARIS.getMillisKeepLocal(LONDON, millisParis));
DateTimeZone zone = DateTimeZone.getDefault();
try {
DateTimeZone.setDefault(LONDON);
assertEquals(millisLondon, PARIS.getMillisKeepLocal(null, millisParis));
} finally {
DateTimeZone.setDefault(zone);
}
}
//-----------------------------------------------------------------------
public void testIsFixed() {
DateTimeZone zone = DateTimeZone.forID("Europe/Paris");
assertEquals(false, zone.isFixed());
assertEquals(true, DateTimeZone.UTC.isFixed());
}
//-----------------------------------------------------------------------
public void testTransitionFixed() {
DateTimeZone zone = DateTimeZone.forID("+01:00");
assertEquals(TEST_TIME_SUMMER, zone.nextTransition(TEST_TIME_SUMMER));
assertEquals(TEST_TIME_WINTER, zone.nextTransition(TEST_TIME_WINTER));
assertEquals(TEST_TIME_SUMMER, zone.previousTransition(TEST_TIME_SUMMER));
assertEquals(TEST_TIME_WINTER, zone.previousTransition(TEST_TIME_WINTER));
}
// //-----------------------------------------------------------------------
// public void testIsLocalDateTimeOverlap_Berlin() {
// DateTimeZone zone = DateTimeZone.forID("Europe/Berlin");
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 10, 28, 1, 0)));
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 10, 28, 1, 59, 59, 99)));
// assertEquals(true, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 10, 28, 2, 0)));
// assertEquals(true, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 10, 28, 2, 30)));
// assertEquals(true, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 10, 28, 2, 59, 59, 99)));
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 10, 28, 3, 0)));
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 10, 28, 4, 0)));
//
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 3, 25, 1, 30))); // before gap
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 3, 25, 2, 30))); // gap
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 3, 25, 3, 30))); // after gap
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 12, 24, 12, 34)));
// }
//
// //-----------------------------------------------------------------------
// public void testIsLocalDateTimeOverlap_NewYork() {
// DateTimeZone zone = DateTimeZone.forID("America/New_York");
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 11, 4, 0, 0)));
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 11, 4, 0, 59, 59, 99)));
// assertEquals(true, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 11, 4, 1, 0)));
// assertEquals(true, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 11, 4, 1, 30)));
// assertEquals(true, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 11, 4, 1, 59, 59, 99)));
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 11, 4, 2, 0)));
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 11, 4, 3, 0)));
//
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 3, 11, 1, 30))); // before gap
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 3, 11, 2, 30))); // gap
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 3, 11, 3, 30))); // after gap
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 12, 24, 12, 34)));
// }
//-----------------------------------------------------------------------
public void testIsLocalDateTimeGap_Berlin() {
DateTimeZone zone = DateTimeZone.forID("Europe/Berlin");
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 25, 1, 0)));
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 25, 1, 59, 59, 99)));
assertEquals(true, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 25, 2, 0)));
assertEquals(true, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 25, 2, 30)));
assertEquals(true, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 25, 2, 59, 59, 99)));
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 25, 3, 0)));
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 25, 4, 0)));
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 10, 28, 1, 30))); // before overlap
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 10, 28, 2, 30))); // overlap
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 10, 28, 3, 30))); // after overlap
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 12, 24, 12, 34)));
}
//-----------------------------------------------------------------------
public void testIsLocalDateTimeGap_NewYork() {
DateTimeZone zone = DateTimeZone.forID("America/New_York");
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 11, 1, 0)));
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 11, 1, 59, 59, 99)));
assertEquals(true, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 11, 2, 0)));
assertEquals(true, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 11, 2, 30)));
assertEquals(true, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 11, 2, 59, 59, 99)));
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 11, 3, 0)));
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 11, 4, 0)));
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 11, 4, 0, 30))); // before overlap
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 11, 4, 1, 30))); // overlap
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 11, 4, 2, 30))); // after overlap
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 12, 24, 12, 34)));
}
//-----------------------------------------------------------------------
public void testToTimeZone() {
DateTimeZone zone = DateTimeZone.forID("Europe/Paris");
TimeZone tz = zone.toTimeZone();
assertEquals("Europe/Paris", tz.getID());
}
//-----------------------------------------------------------------------
public void testEqualsHashCode() {
DateTimeZone zone1 = DateTimeZone.forID("Europe/Paris");
DateTimeZone zone2 = DateTimeZone.forID("Europe/Paris");
assertEquals(true, zone1.equals(zone1));
assertEquals(true, zone1.equals(zone2));
assertEquals(true, zone2.equals(zone1));
assertEquals(true, zone2.equals(zone2));
assertEquals(true, zone1.hashCode() == zone2.hashCode());
DateTimeZone zone3 = DateTimeZone.forID("Europe/London");
assertEquals(true, zone3.equals(zone3));
assertEquals(false, zone1.equals(zone3));
assertEquals(false, zone2.equals(zone3));
assertEquals(false, zone3.equals(zone1));
assertEquals(false, zone3.equals(zone2));
assertEquals(false, zone1.hashCode() == zone3.hashCode());
assertEquals(true, zone3.hashCode() == zone3.hashCode());
DateTimeZone zone4 = DateTimeZone.forID("+01:00");
assertEquals(true, zone4.equals(zone4));
assertEquals(false, zone1.equals(zone4));
assertEquals(false, zone2.equals(zone4));
assertEquals(false, zone3.equals(zone4));
assertEquals(false, zone4.equals(zone1));
assertEquals(false, zone4.equals(zone2));
assertEquals(false, zone4.equals(zone3));
assertEquals(false, zone1.hashCode() == zone4.hashCode());
assertEquals(true, zone4.hashCode() == zone4.hashCode());
DateTimeZone zone5 = DateTimeZone.forID("+02:00");
assertEquals(true, zone5.equals(zone5));
assertEquals(false, zone1.equals(zone5));
assertEquals(false, zone2.equals(zone5));
assertEquals(false, zone3.equals(zone5));
assertEquals(false, zone4.equals(zone5));
assertEquals(false, zone5.equals(zone1));
assertEquals(false, zone5.equals(zone2));
assertEquals(false, zone5.equals(zone3));
assertEquals(false, zone5.equals(zone4));
assertEquals(false, zone1.hashCode() == zone5.hashCode());
assertEquals(true, zone5.hashCode() == zone5.hashCode());
}
//-----------------------------------------------------------------------
public void testToString() {
DateTimeZone zone = DateTimeZone.forID("Europe/Paris");
assertEquals("Europe/Paris", zone.toString());
assertEquals("UTC", DateTimeZone.UTC.toString());
}
//-----------------------------------------------------------------------
public void testSerialization1() throws Exception {
DateTimeZone zone = DateTimeZone.forID("Europe/Paris");
ByteArrayOutputStream baos = new ByteArrayOutputStream();
ObjectOutputStream oos = new ObjectOutputStream(baos);
oos.writeObject(zone);
byte[] bytes = baos.toByteArray();
oos.close();
ByteArrayInputStream bais = new ByteArrayInputStream(bytes);
ObjectInputStream ois = new ObjectInputStream(bais);
DateTimeZone result = (DateTimeZone) ois.readObject();
ois.close();
assertSame(zone, result);
}
//-----------------------------------------------------------------------
public void testSerialization2() throws Exception {
DateTimeZone zone = DateTimeZone.forID("+01:00");
ByteArrayOutputStream baos = new ByteArrayOutputStream();
ObjectOutputStream oos = new ObjectOutputStream(baos);
oos.writeObject(zone);
byte[] bytes = baos.toByteArray();
oos.close();
ByteArrayInputStream bais = new ByteArrayInputStream(bytes);
ObjectInputStream ois = new ObjectInputStream(bais);
DateTimeZone result = (DateTimeZone) ois.readObject();
ois.close();
assertSame(zone, result);
}
public void testCommentParse() throws Exception {
// A bug in ZoneInfoCompiler's handling of comments broke Europe/Athens
// after 1980. This test is included to make sure it doesn't break again.
DateTimeZone zone = DateTimeZone.forID("Europe/Athens");
DateTime dt = new DateTime(2005, 5, 5, 20, 10, 15, 0, zone);
assertEquals(1115313015000L, dt.getMillis());
}
public void testPatchedNameKeysLondon() throws Exception {
// the tz database does not have unique name keys [1716305]
DateTimeZone zone = DateTimeZone.forID("Europe/London");
DateTime now = new DateTime(2007, 1, 1, 0, 0, 0, 0);
String str1 = zone.getName(now.getMillis());
String str2 = zone.getName(now.plusMonths(6).getMillis());
assertEquals(false, str1.equals(str2));
}
public void testPatchedNameKeysSydney() throws Exception {
// the tz database does not have unique name keys [1716305]
DateTimeZone zone = DateTimeZone.forID("Australia/Sydney");
DateTime now = new DateTime(2007, 1, 1, 0, 0, 0, 0);
String str1 = zone.getName(now.getMillis());
String str2 = zone.getName(now.plusMonths(6).getMillis());
assertEquals(false, str1.equals(str2));
}
public void testPatchedNameKeysSydneyHistoric() throws Exception {
// the tz database does not have unique name keys [1716305]
DateTimeZone zone = DateTimeZone.forID("Australia/Sydney");
DateTime now = new DateTime(1996, 1, 1, 0, 0, 0, 0);
String str1 = zone.getName(now.getMillis());
String str2 = zone.getName(now.plusMonths(6).getMillis());
assertEquals(false, str1.equals(str2));
}
public void testPatchedNameKeysGazaHistoric() throws Exception {
// the tz database does not have unique name keys [1716305]
DateTimeZone zone = DateTimeZone.forID("Africa/Johannesburg");
DateTime now = new DateTime(1943, 1, 1, 0, 0, 0, 0);
String str1 = zone.getName(now.getMillis());
String str2 = zone.getName(now.plusMonths(6).getMillis());
assertEquals(false, str1.equals(str2));
}
} | // You are a professional Java test case writer, please create a test case named `testForOffsetHoursMinutes_int_int` for the issue `Time-42`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Time-42
//
// ## Issue-Title:
// DateTimeZone.forOffsetHoursMinutes cannot handle negative offset < 1 hour
//
// ## Issue-Description:
// `DateTimeZone.forOffsetHoursMinutes(h,m)` cannot handle negative offset < 1 hour like `-0:30` due to argument range checking. I used `forOffsetMillis()` instead.
//
//
// This should probably be mentioned in the documentation or negative minutes be accepted.
//
//
//
//
public void testForOffsetHoursMinutes_int_int() {
| 338 | //----------------------------------------------------------------------- | 8 | 302 | src/test/java/org/joda/time/TestDateTimeZone.java | src/test/java | ```markdown
## Issue-ID: Time-42
## Issue-Title:
DateTimeZone.forOffsetHoursMinutes cannot handle negative offset < 1 hour
## Issue-Description:
`DateTimeZone.forOffsetHoursMinutes(h,m)` cannot handle negative offset < 1 hour like `-0:30` due to argument range checking. I used `forOffsetMillis()` instead.
This should probably be mentioned in the documentation or negative minutes be accepted.
```
You are a professional Java test case writer, please create a test case named `testForOffsetHoursMinutes_int_int` for the issue `Time-42`, utilizing the provided issue report information and the following function signature.
```java
public void testForOffsetHoursMinutes_int_int() {
```
| 302 | [
"org.joda.time.DateTimeZone"
] | 7c6c9aae04dcc9c095ba553c27286a63acd78d39f2d419f2d2aeb309788b1c95 | public void testForOffsetHoursMinutes_int_int() | // You are a professional Java test case writer, please create a test case named `testForOffsetHoursMinutes_int_int` for the issue `Time-42`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Time-42
//
// ## Issue-Title:
// DateTimeZone.forOffsetHoursMinutes cannot handle negative offset < 1 hour
//
// ## Issue-Description:
// `DateTimeZone.forOffsetHoursMinutes(h,m)` cannot handle negative offset < 1 hour like `-0:30` due to argument range checking. I used `forOffsetMillis()` instead.
//
//
// This should probably be mentioned in the documentation or negative minutes be accepted.
//
//
//
//
| Time | /*
* Copyright 2001-2013 Stephen Colebourne
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.joda.time;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.io.PrintStream;
import java.lang.reflect.Modifier;
import java.security.AllPermission;
import java.security.CodeSource;
import java.security.Permission;
import java.security.PermissionCollection;
import java.security.Permissions;
import java.security.Policy;
import java.security.ProtectionDomain;
import java.text.DateFormatSymbols;
import java.util.HashSet;
import java.util.LinkedHashMap;
import java.util.Locale;
import java.util.Map;
import java.util.Set;
import java.util.TimeZone;
import junit.framework.TestCase;
import junit.framework.TestSuite;
import org.joda.time.tz.DefaultNameProvider;
import org.joda.time.tz.NameProvider;
import org.joda.time.tz.Provider;
import org.joda.time.tz.UTCProvider;
import org.joda.time.tz.ZoneInfoProvider;
/**
* This class is a JUnit test for DateTimeZone.
*
* @author Stephen Colebourne
*/
public class TestDateTimeZone extends TestCase {
private static final boolean OLD_JDK;
static {
String str = System.getProperty("java.version");
boolean old = true;
if (str.length() > 3 &&
str.charAt(0) == '1' &&
str.charAt(1) == '.' &&
(str.charAt(2) == '4' || str.charAt(2) == '5' || str.charAt(2) == '6')) {
old = false;
}
OLD_JDK = old;
}
// Test in 2002/03 as time zones are more well known
// (before the late 90's they were all over the place)
private static final DateTimeZone PARIS = DateTimeZone.forID("Europe/Paris");
private static final DateTimeZone LONDON = DateTimeZone.forID("Europe/London");
long y2002days = 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 +
365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365;
long y2003days = 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 +
365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365 + 365;
// 2002-06-09
private long TEST_TIME_SUMMER =
(y2002days + 31L + 28L + 31L + 30L + 31L + 9L -1L) * DateTimeConstants.MILLIS_PER_DAY;
// 2002-01-09
private long TEST_TIME_WINTER =
(y2002days + 9L -1L) * DateTimeConstants.MILLIS_PER_DAY;
// // 2002-04-05 Fri
// private long TEST_TIME1 =
// (y2002days + 31L + 28L + 31L + 5L -1L) * DateTimeConstants.MILLIS_PER_DAY
// + 12L * DateTimeConstants.MILLIS_PER_HOUR
// + 24L * DateTimeConstants.MILLIS_PER_MINUTE;
//
// // 2003-05-06 Tue
// private long TEST_TIME2 =
// (y2003days + 31L + 28L + 31L + 30L + 6L -1L) * DateTimeConstants.MILLIS_PER_DAY
// + 14L * DateTimeConstants.MILLIS_PER_HOUR
// + 28L * DateTimeConstants.MILLIS_PER_MINUTE;
private static final Policy RESTRICT;
private static final Policy ALLOW;
static {
// don't call Policy.getPolicy()
RESTRICT = new Policy() {
public PermissionCollection getPermissions(CodeSource codesource) {
Permissions p = new Permissions();
p.add(new AllPermission()); // enable everything
return p;
}
public void refresh() {
}
public boolean implies(ProtectionDomain domain, Permission permission) {
if (permission instanceof JodaTimePermission) {
return false;
}
return true;
// return super.implies(domain, permission);
}
};
ALLOW = new Policy() {
public PermissionCollection getPermissions(CodeSource codesource) {
Permissions p = new Permissions();
p.add(new AllPermission()); // enable everything
return p;
}
public void refresh() {
}
};
}
private DateTimeZone zone;
private Locale locale;
public static void main(String[] args) {
junit.textui.TestRunner.run(suite());
}
public static TestSuite suite() {
return new TestSuite(TestDateTimeZone.class);
}
public TestDateTimeZone(String name) {
super(name);
}
protected void setUp() throws Exception {
locale = Locale.getDefault();
zone = DateTimeZone.getDefault();
Locale.setDefault(Locale.UK);
}
protected void tearDown() throws Exception {
Locale.setDefault(locale);
DateTimeZone.setDefault(zone);
}
//-----------------------------------------------------------------------
public void testDefault() {
assertNotNull(DateTimeZone.getDefault());
DateTimeZone.setDefault(PARIS);
assertSame(PARIS, DateTimeZone.getDefault());
try {
DateTimeZone.setDefault(null);
fail();
} catch (IllegalArgumentException ex) {}
}
public void testDefaultSecurity() {
if (OLD_JDK) {
return;
}
try {
Policy.setPolicy(RESTRICT);
System.setSecurityManager(new SecurityManager());
DateTimeZone.setDefault(PARIS);
fail();
} catch (SecurityException ex) {
// ok
} finally {
System.setSecurityManager(null);
Policy.setPolicy(ALLOW);
}
}
//-----------------------------------------------------------------------
public void testForID_String() {
assertEquals(DateTimeZone.getDefault(), DateTimeZone.forID((String) null));
DateTimeZone zone = DateTimeZone.forID("Europe/London");
assertEquals("Europe/London", zone.getID());
zone = DateTimeZone.forID("UTC");
assertSame(DateTimeZone.UTC, zone);
zone = DateTimeZone.forID("+00:00");
assertSame(DateTimeZone.UTC, zone);
zone = DateTimeZone.forID("+00");
assertSame(DateTimeZone.UTC, zone);
zone = DateTimeZone.forID("+01:23");
assertEquals("+01:23", zone.getID());
assertEquals(DateTimeConstants.MILLIS_PER_HOUR + (23L * DateTimeConstants.MILLIS_PER_MINUTE),
zone.getOffset(TEST_TIME_SUMMER));
zone = DateTimeZone.forID("-02:00");
assertEquals("-02:00", zone.getID());
assertEquals((-2L * DateTimeConstants.MILLIS_PER_HOUR),
zone.getOffset(TEST_TIME_SUMMER));
zone = DateTimeZone.forID("-07:05:34.0");
assertEquals("-07:05:34", zone.getID());
assertEquals((-7L * DateTimeConstants.MILLIS_PER_HOUR) +
(-5L * DateTimeConstants.MILLIS_PER_MINUTE) +
(-34L * DateTimeConstants.MILLIS_PER_SECOND),
zone.getOffset(TEST_TIME_SUMMER));
try {
DateTimeZone.forID("SST");
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.forID("europe/london");
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.forID("Europe/UK");
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.forID("+");
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.forID("+0");
fail();
} catch (IllegalArgumentException ex) {}
}
public void testForID_String_old() {
Map<String, String> map = new LinkedHashMap<String, String>();
map.put("GMT", "UTC");
map.put("WET", "WET");
map.put("CET", "CET");
map.put("MET", "CET");
map.put("ECT", "CET");
map.put("EET", "EET");
map.put("MIT", "Pacific/Apia");
map.put("HST", "Pacific/Honolulu");
map.put("AST", "America/Anchorage");
map.put("PST", "America/Los_Angeles");
map.put("MST", "America/Denver");
map.put("PNT", "America/Phoenix");
map.put("CST", "America/Chicago");
map.put("EST", "America/New_York");
map.put("IET", "America/Indiana/Indianapolis");
map.put("PRT", "America/Puerto_Rico");
map.put("CNT", "America/St_Johns");
map.put("AGT", "America/Argentina/Buenos_Aires");
map.put("BET", "America/Sao_Paulo");
map.put("ART", "Africa/Cairo");
map.put("CAT", "Africa/Harare");
map.put("EAT", "Africa/Addis_Ababa");
map.put("NET", "Asia/Yerevan");
map.put("PLT", "Asia/Karachi");
map.put("IST", "Asia/Kolkata");
map.put("BST", "Asia/Dhaka");
map.put("VST", "Asia/Ho_Chi_Minh");
map.put("CTT", "Asia/Shanghai");
map.put("JST", "Asia/Tokyo");
map.put("ACT", "Australia/Darwin");
map.put("AET", "Australia/Sydney");
map.put("SST", "Pacific/Guadalcanal");
map.put("NST", "Pacific/Auckland");
for (String key : map.keySet()) {
String value = map.get(key);
TimeZone juZone = TimeZone.getTimeZone(key);
DateTimeZone zone = DateTimeZone.forTimeZone(juZone);
assertEquals(value, zone.getID());
// System.out.println(juZone);
// System.out.println(juZone.getDisplayName());
// System.out.println(zone);
// System.out.println("------");
}
}
//-----------------------------------------------------------------------
public void testForOffsetHours_int() {
assertEquals(DateTimeZone.UTC, DateTimeZone.forOffsetHours(0));
assertEquals(DateTimeZone.forID("+03:00"), DateTimeZone.forOffsetHours(3));
assertEquals(DateTimeZone.forID("-02:00"), DateTimeZone.forOffsetHours(-2));
try {
DateTimeZone.forOffsetHours(999999);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testForOffsetHoursMinutes_int_int() {
assertEquals(DateTimeZone.UTC, DateTimeZone.forOffsetHoursMinutes(0, 0));
assertEquals(DateTimeZone.forID("+23:59"), DateTimeZone.forOffsetHoursMinutes(23, 59));
assertEquals(DateTimeZone.forID("+02:15"), DateTimeZone.forOffsetHoursMinutes(2, 15));
assertEquals(DateTimeZone.forID("+02:00"), DateTimeZone.forOffsetHoursMinutes(2, 0));
try {
DateTimeZone.forOffsetHoursMinutes(2, -15);
fail();
} catch (IllegalArgumentException ex) {}
assertEquals(DateTimeZone.forID("+00:15"), DateTimeZone.forOffsetHoursMinutes(0, 15));
assertEquals(DateTimeZone.forID("+00:00"), DateTimeZone.forOffsetHoursMinutes(0, 0));
assertEquals(DateTimeZone.forID("-00:15"), DateTimeZone.forOffsetHoursMinutes(0, -15));
assertEquals(DateTimeZone.forID("-02:00"), DateTimeZone.forOffsetHoursMinutes(-2, 0));
assertEquals(DateTimeZone.forID("-02:15"), DateTimeZone.forOffsetHoursMinutes(-2, -15));
assertEquals(DateTimeZone.forID("-02:15"), DateTimeZone.forOffsetHoursMinutes(-2, 15));
assertEquals(DateTimeZone.forID("-23:59"), DateTimeZone.forOffsetHoursMinutes(-23, 59));
try {
DateTimeZone.forOffsetHoursMinutes(2, 60);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.forOffsetHoursMinutes(-2, 60);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.forOffsetHoursMinutes(24, 0);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.forOffsetHoursMinutes(-24, 0);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testForOffsetMillis_int() {
assertSame(DateTimeZone.UTC, DateTimeZone.forOffsetMillis(0));
assertEquals(DateTimeZone.forID("+23:59:59.999"), DateTimeZone.forOffsetMillis((24 * 60 * 60 * 1000) - 1));
assertEquals(DateTimeZone.forID("+03:00"), DateTimeZone.forOffsetMillis(3 * 60 * 60 * 1000));
assertEquals(DateTimeZone.forID("-02:00"), DateTimeZone.forOffsetMillis(-2 * 60 * 60 * 1000));
assertEquals(DateTimeZone.forID("-23:59:59.999"), DateTimeZone.forOffsetMillis((-24 * 60 * 60 * 1000) + 1));
assertEquals(DateTimeZone.forID("+04:45:17.045"),
DateTimeZone.forOffsetMillis(
4 * 60 * 60 * 1000 + 45 * 60 * 1000 + 17 * 1000 + 45));
}
//-----------------------------------------------------------------------
public void testForTimeZone_TimeZone() {
assertEquals(DateTimeZone.getDefault(), DateTimeZone.forTimeZone((TimeZone) null));
DateTimeZone zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("Europe/London"));
assertEquals("Europe/London", zone.getID());
assertSame(DateTimeZone.UTC, DateTimeZone.forTimeZone(TimeZone.getTimeZone("UTC")));
zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("+00:00"));
assertSame(DateTimeZone.UTC, zone);
zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("GMT+00:00"));
assertSame(DateTimeZone.UTC, zone);
zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("GMT+00:00"));
assertSame(DateTimeZone.UTC, zone);
zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("GMT+00"));
assertSame(DateTimeZone.UTC, zone);
zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("GMT+01:23"));
assertEquals("+01:23", zone.getID());
assertEquals(DateTimeConstants.MILLIS_PER_HOUR + (23L * DateTimeConstants.MILLIS_PER_MINUTE),
zone.getOffset(TEST_TIME_SUMMER));
zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("GMT+1:23"));
assertEquals("+01:23", zone.getID());
assertEquals(DateTimeConstants.MILLIS_PER_HOUR + (23L * DateTimeConstants.MILLIS_PER_MINUTE),
zone.getOffset(TEST_TIME_SUMMER));
zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("GMT-02:00"));
assertEquals("-02:00", zone.getID());
assertEquals((-2L * DateTimeConstants.MILLIS_PER_HOUR), zone.getOffset(TEST_TIME_SUMMER));
zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("GMT+2"));
assertEquals("+02:00", zone.getID());
assertEquals((2L * DateTimeConstants.MILLIS_PER_HOUR), zone.getOffset(TEST_TIME_SUMMER));
zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("EST"));
assertEquals("America/New_York", zone.getID());
}
public void testTimeZoneConversion() {
TimeZone jdkTimeZone = TimeZone.getTimeZone("GMT-10");
assertEquals("GMT-10:00", jdkTimeZone.getID());
DateTimeZone jodaTimeZone = DateTimeZone.forTimeZone(jdkTimeZone);
assertEquals("-10:00", jodaTimeZone.getID());
assertEquals(jdkTimeZone.getRawOffset(), jodaTimeZone.getOffset(0L));
TimeZone convertedTimeZone = jodaTimeZone.toTimeZone();
assertEquals("GMT-10:00", jdkTimeZone.getID());
assertEquals(jdkTimeZone.getID(), convertedTimeZone.getID());
assertEquals(jdkTimeZone.getRawOffset(), convertedTimeZone.getRawOffset());
}
//-----------------------------------------------------------------------
public void testGetAvailableIDs() {
assertTrue(DateTimeZone.getAvailableIDs().contains("UTC"));
}
//-----------------------------------------------------------------------
public void testProvider() {
try {
assertNotNull(DateTimeZone.getProvider());
Provider provider = DateTimeZone.getProvider();
DateTimeZone.setProvider(null);
assertEquals(provider.getClass(), DateTimeZone.getProvider().getClass());
try {
DateTimeZone.setProvider(new MockNullIDSProvider());
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.setProvider(new MockEmptyIDSProvider());
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.setProvider(new MockNoUTCProvider());
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.setProvider(new MockBadUTCProvider());
fail();
} catch (IllegalArgumentException ex) {}
Provider prov = new MockOKProvider();
DateTimeZone.setProvider(prov);
assertSame(prov, DateTimeZone.getProvider());
assertEquals(2, DateTimeZone.getAvailableIDs().size());
assertTrue(DateTimeZone.getAvailableIDs().contains("UTC"));
assertTrue(DateTimeZone.getAvailableIDs().contains("Europe/London"));
} finally {
DateTimeZone.setProvider(null);
assertEquals(ZoneInfoProvider.class, DateTimeZone.getProvider().getClass());
}
try {
System.setProperty("org.joda.time.DateTimeZone.Provider", "org.joda.time.tz.UTCProvider");
DateTimeZone.setProvider(null);
assertEquals(UTCProvider.class, DateTimeZone.getProvider().getClass());
} finally {
System.getProperties().remove("org.joda.time.DateTimeZone.Provider");
DateTimeZone.setProvider(null);
assertEquals(ZoneInfoProvider.class, DateTimeZone.getProvider().getClass());
}
PrintStream syserr = System.err;
try {
System.setProperty("org.joda.time.DateTimeZone.Provider", "xxx");
ByteArrayOutputStream baos = new ByteArrayOutputStream();
System.setErr(new PrintStream(baos));
DateTimeZone.setProvider(null);
assertEquals(ZoneInfoProvider.class, DateTimeZone.getProvider().getClass());
String str = new String(baos.toByteArray());
assertTrue(str.indexOf("java.lang.ClassNotFoundException") >= 0);
} finally {
System.setErr(syserr);
System.getProperties().remove("org.joda.time.DateTimeZone.Provider");
DateTimeZone.setProvider(null);
assertEquals(ZoneInfoProvider.class, DateTimeZone.getProvider().getClass());
}
}
public void testProviderSecurity() {
if (OLD_JDK) {
return;
}
try {
Policy.setPolicy(RESTRICT);
System.setSecurityManager(new SecurityManager());
DateTimeZone.setProvider(new MockOKProvider());
fail();
} catch (SecurityException ex) {
// ok
} finally {
System.setSecurityManager(null);
Policy.setPolicy(ALLOW);
}
}
static class MockNullIDSProvider implements Provider {
public Set getAvailableIDs() {
return null;
}
public DateTimeZone getZone(String id) {
return null;
}
}
static class MockEmptyIDSProvider implements Provider {
public Set getAvailableIDs() {
return new HashSet();
}
public DateTimeZone getZone(String id) {
return null;
}
}
static class MockNoUTCProvider implements Provider {
public Set getAvailableIDs() {
Set set = new HashSet();
set.add("Europe/London");
return set;
}
public DateTimeZone getZone(String id) {
return null;
}
}
static class MockBadUTCProvider implements Provider {
public Set getAvailableIDs() {
Set set = new HashSet();
set.add("UTC");
set.add("Europe/London");
return set;
}
public DateTimeZone getZone(String id) {
return null;
}
}
static class MockOKProvider implements Provider {
public Set getAvailableIDs() {
Set set = new HashSet();
set.add("UTC");
set.add("Europe/London");
return set;
}
public DateTimeZone getZone(String id) {
return DateTimeZone.UTC;
}
}
//-----------------------------------------------------------------------
public void testNameProvider() {
try {
assertNotNull(DateTimeZone.getNameProvider());
NameProvider provider = DateTimeZone.getNameProvider();
DateTimeZone.setNameProvider(null);
assertEquals(provider.getClass(), DateTimeZone.getNameProvider().getClass());
provider = new MockOKButNullNameProvider();
DateTimeZone.setNameProvider(provider);
assertSame(provider, DateTimeZone.getNameProvider());
assertEquals("+00:00", DateTimeZone.UTC.getShortName(TEST_TIME_SUMMER));
assertEquals("+00:00", DateTimeZone.UTC.getName(TEST_TIME_SUMMER));
} finally {
DateTimeZone.setNameProvider(null);
}
try {
System.setProperty("org.joda.time.DateTimeZone.NameProvider", "org.joda.time.tz.DefaultNameProvider");
DateTimeZone.setNameProvider(null);
assertEquals(DefaultNameProvider.class, DateTimeZone.getNameProvider().getClass());
} finally {
System.getProperties().remove("org.joda.time.DateTimeZone.NameProvider");
DateTimeZone.setNameProvider(null);
assertEquals(DefaultNameProvider.class, DateTimeZone.getNameProvider().getClass());
}
PrintStream syserr = System.err;
try {
System.setProperty("org.joda.time.DateTimeZone.NameProvider", "xxx");
ByteArrayOutputStream baos = new ByteArrayOutputStream();
System.setErr(new PrintStream(baos));
DateTimeZone.setNameProvider(null);
assertEquals(DefaultNameProvider.class, DateTimeZone.getNameProvider().getClass());
String str = new String(baos.toByteArray());
assertTrue(str.indexOf("java.lang.ClassNotFoundException") >= 0);
} finally {
System.setErr(syserr);
System.getProperties().remove("org.joda.time.DateTimeZone.NameProvider");
DateTimeZone.setNameProvider(null);
assertEquals(DefaultNameProvider.class, DateTimeZone.getNameProvider().getClass());
}
}
public void testNameProviderSecurity() {
if (OLD_JDK) {
return;
}
try {
Policy.setPolicy(RESTRICT);
System.setSecurityManager(new SecurityManager());
DateTimeZone.setNameProvider(new MockOKButNullNameProvider());
fail();
} catch (SecurityException ex) {
// ok
} finally {
System.setSecurityManager(null);
Policy.setPolicy(ALLOW);
}
}
static class MockOKButNullNameProvider implements NameProvider {
public String getShortName(Locale locale, String id, String nameKey) {
return null;
}
public String getName(Locale locale, String id, String nameKey) {
return null;
}
}
//-----------------------------------------------------------------------
public void testConstructor() {
assertEquals(1, DateTimeZone.class.getDeclaredConstructors().length);
assertTrue(Modifier.isProtected(DateTimeZone.class.getDeclaredConstructors()[0].getModifiers()));
try {
new DateTimeZone(null) {
public String getNameKey(long instant) {
return null;
}
public int getOffset(long instant) {
return 0;
}
public int getStandardOffset(long instant) {
return 0;
}
public boolean isFixed() {
return false;
}
public long nextTransition(long instant) {
return 0;
}
public long previousTransition(long instant) {
return 0;
}
public boolean equals(Object object) {
return false;
}
};
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testGetID() {
DateTimeZone zone = DateTimeZone.forID("Europe/Paris");
assertEquals("Europe/Paris", zone.getID());
}
public void testGetNameKey() {
DateTimeZone zone = DateTimeZone.forID("Europe/London");
assertEquals("BST", zone.getNameKey(TEST_TIME_SUMMER));
assertEquals("GMT", zone.getNameKey(TEST_TIME_WINTER));
}
static final boolean JDK6;
static {
boolean jdk6 = true;
try {
DateFormatSymbols.class.getMethod("getInstance", new Class[] {Locale.class});
} catch (Exception ex) {
jdk6 = false;
}
JDK6 = jdk6;
}
public void testGetShortName() {}
// Defects4J: flaky method
// public void testGetShortName() {
// DateTimeZone zone = DateTimeZone.forID("Europe/London");
// assertEquals("BST", zone.getShortName(TEST_TIME_SUMMER));
// assertEquals("GMT", zone.getShortName(TEST_TIME_WINTER));
// assertEquals("BST", zone.getShortName(TEST_TIME_SUMMER, Locale.ENGLISH));
// }
public void testGetShortName_berlin() {}
// Defects4J: flaky method
// public void testGetShortName_berlin() {
// DateTimeZone berlin = DateTimeZone.forID("Europe/Berlin");
// assertEquals("CET", berlin.getShortName(TEST_TIME_WINTER, Locale.ENGLISH));
// assertEquals("CEST", berlin.getShortName(TEST_TIME_SUMMER, Locale.ENGLISH));
// if (JDK6) {
// assertEquals("MEZ", berlin.getShortName(TEST_TIME_WINTER, Locale.GERMAN));
// assertEquals("MESZ", berlin.getShortName(TEST_TIME_SUMMER, Locale.GERMAN));
// } else {
// assertEquals("CET", berlin.getShortName(TEST_TIME_WINTER, Locale.GERMAN));
// assertEquals("CEST", berlin.getShortName(TEST_TIME_SUMMER, Locale.GERMAN));
// }
// }
public void testGetShortNameProviderName() {
assertEquals(null, DateTimeZone.getNameProvider().getShortName(null, "Europe/London", "BST"));
assertEquals(null, DateTimeZone.getNameProvider().getShortName(Locale.ENGLISH, null, "BST"));
assertEquals(null, DateTimeZone.getNameProvider().getShortName(Locale.ENGLISH, "Europe/London", null));
assertEquals(null, DateTimeZone.getNameProvider().getShortName(null, null, null));
}
public void testGetShortNameNullKey() {
DateTimeZone zone = new MockDateTimeZone("Europe/London");
assertEquals("Europe/London", zone.getShortName(TEST_TIME_SUMMER, Locale.ENGLISH));
}
public void testGetName() {}
// Defects4J: flaky method
// public void testGetName() {
// DateTimeZone zone = DateTimeZone.forID("Europe/London");
// assertEquals("British Summer Time", zone.getName(TEST_TIME_SUMMER));
// assertEquals("Greenwich Mean Time", zone.getName(TEST_TIME_WINTER));
// assertEquals("British Summer Time", zone.getName(TEST_TIME_SUMMER, Locale.ENGLISH));
// }
public void testGetName_berlin() {}
// Defects4J: flaky method
// public void testGetName_berlin() {
// DateTimeZone berlin = DateTimeZone.forID("Europe/Berlin");
// assertEquals("Central European Time", berlin.getName(TEST_TIME_WINTER, Locale.ENGLISH));
// assertEquals("Central European Summer Time", berlin.getName(TEST_TIME_SUMMER, Locale.ENGLISH));
// if (JDK6) {
// assertEquals("Mitteleurop\u00e4ische Zeit", berlin.getName(TEST_TIME_WINTER, Locale.GERMAN));
// assertEquals("Mitteleurop\u00e4ische Sommerzeit", berlin.getName(TEST_TIME_SUMMER, Locale.GERMAN));
// } else {
// assertEquals("Zentraleurop\u00e4ische Zeit", berlin.getName(TEST_TIME_WINTER, Locale.GERMAN));
// assertEquals("Zentraleurop\u00e4ische Sommerzeit", berlin.getName(TEST_TIME_SUMMER, Locale.GERMAN));
// }
// }
public void testGetNameProviderName() {
assertEquals(null, DateTimeZone.getNameProvider().getName(null, "Europe/London", "BST"));
assertEquals(null, DateTimeZone.getNameProvider().getName(Locale.ENGLISH, null, "BST"));
assertEquals(null, DateTimeZone.getNameProvider().getName(Locale.ENGLISH, "Europe/London", null));
assertEquals(null, DateTimeZone.getNameProvider().getName(null, null, null));
}
public void testGetNameNullKey() {
DateTimeZone zone = new MockDateTimeZone("Europe/London");
assertEquals("Europe/London", zone.getName(TEST_TIME_SUMMER, Locale.ENGLISH));
}
static class MockDateTimeZone extends DateTimeZone {
public MockDateTimeZone(String id) {
super(id);
}
public String getNameKey(long instant) {
return null; // null
}
public int getOffset(long instant) {
return 0;
}
public int getStandardOffset(long instant) {
return 0;
}
public boolean isFixed() {
return false;
}
public long nextTransition(long instant) {
return 0;
}
public long previousTransition(long instant) {
return 0;
}
public boolean equals(Object object) {
return false;
}
}
//-----------------------------------------------------------------------
public void testGetOffset_long() {
DateTimeZone zone = DateTimeZone.forID("Europe/Paris");
assertEquals(2L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffset(TEST_TIME_SUMMER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffset(TEST_TIME_WINTER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getStandardOffset(TEST_TIME_SUMMER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getStandardOffset(TEST_TIME_WINTER));
assertEquals(2L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffsetFromLocal(TEST_TIME_SUMMER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffsetFromLocal(TEST_TIME_WINTER));
assertEquals(false, zone.isStandardOffset(TEST_TIME_SUMMER));
assertEquals(true, zone.isStandardOffset(TEST_TIME_WINTER));
}
public void testGetOffset_RI() {
DateTimeZone zone = DateTimeZone.forID("Europe/Paris");
assertEquals(2L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffset(new Instant(TEST_TIME_SUMMER)));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffset(new Instant(TEST_TIME_WINTER)));
assertEquals(zone.getOffset(DateTimeUtils.currentTimeMillis()), zone.getOffset(null));
}
public void testGetOffsetFixed() {
DateTimeZone zone = DateTimeZone.forID("+01:00");
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffset(TEST_TIME_SUMMER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffset(TEST_TIME_WINTER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getStandardOffset(TEST_TIME_SUMMER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getStandardOffset(TEST_TIME_WINTER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffsetFromLocal(TEST_TIME_SUMMER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffsetFromLocal(TEST_TIME_WINTER));
assertEquals(true, zone.isStandardOffset(TEST_TIME_SUMMER));
assertEquals(true, zone.isStandardOffset(TEST_TIME_WINTER));
}
public void testGetOffsetFixed_RI() {
DateTimeZone zone = DateTimeZone.forID("+01:00");
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffset(new Instant(TEST_TIME_SUMMER)));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffset(new Instant(TEST_TIME_WINTER)));
assertEquals(zone.getOffset(DateTimeUtils.currentTimeMillis()), zone.getOffset(null));
}
//-----------------------------------------------------------------------
public void testGetMillisKeepLocal() {
long millisLondon = TEST_TIME_SUMMER;
long millisParis = TEST_TIME_SUMMER - 1L * DateTimeConstants.MILLIS_PER_HOUR;
assertEquals(millisLondon, LONDON.getMillisKeepLocal(LONDON, millisLondon));
assertEquals(millisParis, LONDON.getMillisKeepLocal(LONDON, millisParis));
assertEquals(millisLondon, PARIS.getMillisKeepLocal(PARIS, millisLondon));
assertEquals(millisParis, PARIS.getMillisKeepLocal(PARIS, millisParis));
assertEquals(millisParis, LONDON.getMillisKeepLocal(PARIS, millisLondon));
assertEquals(millisLondon, PARIS.getMillisKeepLocal(LONDON, millisParis));
DateTimeZone zone = DateTimeZone.getDefault();
try {
DateTimeZone.setDefault(LONDON);
assertEquals(millisLondon, PARIS.getMillisKeepLocal(null, millisParis));
} finally {
DateTimeZone.setDefault(zone);
}
}
//-----------------------------------------------------------------------
public void testIsFixed() {
DateTimeZone zone = DateTimeZone.forID("Europe/Paris");
assertEquals(false, zone.isFixed());
assertEquals(true, DateTimeZone.UTC.isFixed());
}
//-----------------------------------------------------------------------
public void testTransitionFixed() {
DateTimeZone zone = DateTimeZone.forID("+01:00");
assertEquals(TEST_TIME_SUMMER, zone.nextTransition(TEST_TIME_SUMMER));
assertEquals(TEST_TIME_WINTER, zone.nextTransition(TEST_TIME_WINTER));
assertEquals(TEST_TIME_SUMMER, zone.previousTransition(TEST_TIME_SUMMER));
assertEquals(TEST_TIME_WINTER, zone.previousTransition(TEST_TIME_WINTER));
}
// //-----------------------------------------------------------------------
// public void testIsLocalDateTimeOverlap_Berlin() {
// DateTimeZone zone = DateTimeZone.forID("Europe/Berlin");
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 10, 28, 1, 0)));
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 10, 28, 1, 59, 59, 99)));
// assertEquals(true, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 10, 28, 2, 0)));
// assertEquals(true, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 10, 28, 2, 30)));
// assertEquals(true, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 10, 28, 2, 59, 59, 99)));
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 10, 28, 3, 0)));
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 10, 28, 4, 0)));
//
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 3, 25, 1, 30))); // before gap
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 3, 25, 2, 30))); // gap
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 3, 25, 3, 30))); // after gap
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 12, 24, 12, 34)));
// }
//
// //-----------------------------------------------------------------------
// public void testIsLocalDateTimeOverlap_NewYork() {
// DateTimeZone zone = DateTimeZone.forID("America/New_York");
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 11, 4, 0, 0)));
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 11, 4, 0, 59, 59, 99)));
// assertEquals(true, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 11, 4, 1, 0)));
// assertEquals(true, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 11, 4, 1, 30)));
// assertEquals(true, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 11, 4, 1, 59, 59, 99)));
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 11, 4, 2, 0)));
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 11, 4, 3, 0)));
//
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 3, 11, 1, 30))); // before gap
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 3, 11, 2, 30))); // gap
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 3, 11, 3, 30))); // after gap
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 12, 24, 12, 34)));
// }
//-----------------------------------------------------------------------
public void testIsLocalDateTimeGap_Berlin() {
DateTimeZone zone = DateTimeZone.forID("Europe/Berlin");
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 25, 1, 0)));
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 25, 1, 59, 59, 99)));
assertEquals(true, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 25, 2, 0)));
assertEquals(true, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 25, 2, 30)));
assertEquals(true, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 25, 2, 59, 59, 99)));
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 25, 3, 0)));
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 25, 4, 0)));
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 10, 28, 1, 30))); // before overlap
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 10, 28, 2, 30))); // overlap
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 10, 28, 3, 30))); // after overlap
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 12, 24, 12, 34)));
}
//-----------------------------------------------------------------------
public void testIsLocalDateTimeGap_NewYork() {
DateTimeZone zone = DateTimeZone.forID("America/New_York");
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 11, 1, 0)));
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 11, 1, 59, 59, 99)));
assertEquals(true, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 11, 2, 0)));
assertEquals(true, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 11, 2, 30)));
assertEquals(true, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 11, 2, 59, 59, 99)));
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 11, 3, 0)));
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 11, 4, 0)));
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 11, 4, 0, 30))); // before overlap
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 11, 4, 1, 30))); // overlap
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 11, 4, 2, 30))); // after overlap
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 12, 24, 12, 34)));
}
//-----------------------------------------------------------------------
public void testToTimeZone() {
DateTimeZone zone = DateTimeZone.forID("Europe/Paris");
TimeZone tz = zone.toTimeZone();
assertEquals("Europe/Paris", tz.getID());
}
//-----------------------------------------------------------------------
public void testEqualsHashCode() {
DateTimeZone zone1 = DateTimeZone.forID("Europe/Paris");
DateTimeZone zone2 = DateTimeZone.forID("Europe/Paris");
assertEquals(true, zone1.equals(zone1));
assertEquals(true, zone1.equals(zone2));
assertEquals(true, zone2.equals(zone1));
assertEquals(true, zone2.equals(zone2));
assertEquals(true, zone1.hashCode() == zone2.hashCode());
DateTimeZone zone3 = DateTimeZone.forID("Europe/London");
assertEquals(true, zone3.equals(zone3));
assertEquals(false, zone1.equals(zone3));
assertEquals(false, zone2.equals(zone3));
assertEquals(false, zone3.equals(zone1));
assertEquals(false, zone3.equals(zone2));
assertEquals(false, zone1.hashCode() == zone3.hashCode());
assertEquals(true, zone3.hashCode() == zone3.hashCode());
DateTimeZone zone4 = DateTimeZone.forID("+01:00");
assertEquals(true, zone4.equals(zone4));
assertEquals(false, zone1.equals(zone4));
assertEquals(false, zone2.equals(zone4));
assertEquals(false, zone3.equals(zone4));
assertEquals(false, zone4.equals(zone1));
assertEquals(false, zone4.equals(zone2));
assertEquals(false, zone4.equals(zone3));
assertEquals(false, zone1.hashCode() == zone4.hashCode());
assertEquals(true, zone4.hashCode() == zone4.hashCode());
DateTimeZone zone5 = DateTimeZone.forID("+02:00");
assertEquals(true, zone5.equals(zone5));
assertEquals(false, zone1.equals(zone5));
assertEquals(false, zone2.equals(zone5));
assertEquals(false, zone3.equals(zone5));
assertEquals(false, zone4.equals(zone5));
assertEquals(false, zone5.equals(zone1));
assertEquals(false, zone5.equals(zone2));
assertEquals(false, zone5.equals(zone3));
assertEquals(false, zone5.equals(zone4));
assertEquals(false, zone1.hashCode() == zone5.hashCode());
assertEquals(true, zone5.hashCode() == zone5.hashCode());
}
//-----------------------------------------------------------------------
public void testToString() {
DateTimeZone zone = DateTimeZone.forID("Europe/Paris");
assertEquals("Europe/Paris", zone.toString());
assertEquals("UTC", DateTimeZone.UTC.toString());
}
//-----------------------------------------------------------------------
public void testSerialization1() throws Exception {
DateTimeZone zone = DateTimeZone.forID("Europe/Paris");
ByteArrayOutputStream baos = new ByteArrayOutputStream();
ObjectOutputStream oos = new ObjectOutputStream(baos);
oos.writeObject(zone);
byte[] bytes = baos.toByteArray();
oos.close();
ByteArrayInputStream bais = new ByteArrayInputStream(bytes);
ObjectInputStream ois = new ObjectInputStream(bais);
DateTimeZone result = (DateTimeZone) ois.readObject();
ois.close();
assertSame(zone, result);
}
//-----------------------------------------------------------------------
public void testSerialization2() throws Exception {
DateTimeZone zone = DateTimeZone.forID("+01:00");
ByteArrayOutputStream baos = new ByteArrayOutputStream();
ObjectOutputStream oos = new ObjectOutputStream(baos);
oos.writeObject(zone);
byte[] bytes = baos.toByteArray();
oos.close();
ByteArrayInputStream bais = new ByteArrayInputStream(bytes);
ObjectInputStream ois = new ObjectInputStream(bais);
DateTimeZone result = (DateTimeZone) ois.readObject();
ois.close();
assertSame(zone, result);
}
public void testCommentParse() throws Exception {
// A bug in ZoneInfoCompiler's handling of comments broke Europe/Athens
// after 1980. This test is included to make sure it doesn't break again.
DateTimeZone zone = DateTimeZone.forID("Europe/Athens");
DateTime dt = new DateTime(2005, 5, 5, 20, 10, 15, 0, zone);
assertEquals(1115313015000L, dt.getMillis());
}
public void testPatchedNameKeysLondon() throws Exception {
// the tz database does not have unique name keys [1716305]
DateTimeZone zone = DateTimeZone.forID("Europe/London");
DateTime now = new DateTime(2007, 1, 1, 0, 0, 0, 0);
String str1 = zone.getName(now.getMillis());
String str2 = zone.getName(now.plusMonths(6).getMillis());
assertEquals(false, str1.equals(str2));
}
public void testPatchedNameKeysSydney() throws Exception {
// the tz database does not have unique name keys [1716305]
DateTimeZone zone = DateTimeZone.forID("Australia/Sydney");
DateTime now = new DateTime(2007, 1, 1, 0, 0, 0, 0);
String str1 = zone.getName(now.getMillis());
String str2 = zone.getName(now.plusMonths(6).getMillis());
assertEquals(false, str1.equals(str2));
}
public void testPatchedNameKeysSydneyHistoric() throws Exception {
// the tz database does not have unique name keys [1716305]
DateTimeZone zone = DateTimeZone.forID("Australia/Sydney");
DateTime now = new DateTime(1996, 1, 1, 0, 0, 0, 0);
String str1 = zone.getName(now.getMillis());
String str2 = zone.getName(now.plusMonths(6).getMillis());
assertEquals(false, str1.equals(str2));
}
public void testPatchedNameKeysGazaHistoric() throws Exception {
// the tz database does not have unique name keys [1716305]
DateTimeZone zone = DateTimeZone.forID("Africa/Johannesburg");
DateTime now = new DateTime(1943, 1, 1, 0, 0, 0, 0);
String str1 = zone.getName(now.getMillis());
String str2 = zone.getName(now.plusMonths(6).getMillis());
assertEquals(false, str1.equals(str2));
}
} |
|
@Test public void testClonesClassnames() {
Document doc = Jsoup.parse("<div class='one two'></div>");
Element div = doc.select("div").first();
Set<String> classes = div.classNames();
assertEquals(2, classes.size());
assertTrue(classes.contains("one"));
assertTrue(classes.contains("two"));
Element copy = div.clone();
Set<String> copyClasses = copy.classNames();
assertEquals(2, copyClasses.size());
assertTrue(copyClasses.contains("one"));
assertTrue(copyClasses.contains("two"));
copyClasses.add("three");
copyClasses.remove("one");
assertTrue(classes.contains("one"));
assertFalse(classes.contains("three"));
assertFalse(copyClasses.contains("one"));
assertTrue(copyClasses.contains("three"));
assertEquals("", div.html());
assertEquals("", copy.html());
} | org.jsoup.nodes.ElementTest::testClonesClassnames | src/test/java/org/jsoup/nodes/ElementTest.java | 499 | src/test/java/org/jsoup/nodes/ElementTest.java | testClonesClassnames | package org.jsoup.nodes;
import org.jsoup.Jsoup;
import org.jsoup.TextUtil;
import org.jsoup.helper.StringUtil;
import org.jsoup.parser.Tag;
import org.jsoup.select.Elements;
import org.junit.Test;
import static org.junit.Assert.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Set;
import java.util.Map;
/**
* Tests for Element (DOM stuff mostly).
*
* @author Jonathan Hedley
*/
public class ElementTest {
private String reference = "<div id=div1><p>Hello</p><p>Another <b>element</b></p><div id=div2><img src=foo.png></div></div>";
@Test public void getElementsByTagName() {
Document doc = Jsoup.parse(reference);
List<Element> divs = doc.getElementsByTag("div");
assertEquals(2, divs.size());
assertEquals("div1", divs.get(0).id());
assertEquals("div2", divs.get(1).id());
List<Element> ps = doc.getElementsByTag("p");
assertEquals(2, ps.size());
assertEquals("Hello", ((TextNode) ps.get(0).childNode(0)).getWholeText());
assertEquals("Another ", ((TextNode) ps.get(1).childNode(0)).getWholeText());
List<Element> ps2 = doc.getElementsByTag("P");
assertEquals(ps, ps2);
List<Element> imgs = doc.getElementsByTag("img");
assertEquals("foo.png", imgs.get(0).attr("src"));
List<Element> empty = doc.getElementsByTag("wtf");
assertEquals(0, empty.size());
}
@Test public void getNamespacedElementsByTag() {
Document doc = Jsoup.parse("<div><abc:def id=1>Hello</abc:def></div>");
Elements els = doc.getElementsByTag("abc:def");
assertEquals(1, els.size());
assertEquals("1", els.first().id());
assertEquals("abc:def", els.first().tagName());
}
@Test public void testGetElementById() {
Document doc = Jsoup.parse(reference);
Element div = doc.getElementById("div1");
assertEquals("div1", div.id());
assertNull(doc.getElementById("none"));
Document doc2 = Jsoup.parse("<div id=1><div id=2><p>Hello <span id=2>world!</span></p></div></div>");
Element div2 = doc2.getElementById("2");
assertEquals("div", div2.tagName()); // not the span
Element span = div2.child(0).getElementById("2"); // called from <p> context should be span
assertEquals("span", span.tagName());
}
@Test public void testGetText() {
Document doc = Jsoup.parse(reference);
assertEquals("Hello Another element", doc.text());
assertEquals("Another element", doc.getElementsByTag("p").get(1).text());
}
@Test public void testGetChildText() {
Document doc = Jsoup.parse("<p>Hello <b>there</b> now");
Element p = doc.select("p").first();
assertEquals("Hello there now", p.text());
assertEquals("Hello now", p.ownText());
}
@Test public void testNormalisesText() {
String h = "<p>Hello<p>There.</p> \n <p>Here <b>is</b> \n s<b>om</b>e text.";
Document doc = Jsoup.parse(h);
String text = doc.text();
assertEquals("Hello There. Here is some text.", text);
}
@Test public void testKeepsPreText() {
String h = "<p>Hello \n \n there.</p> <div><pre> What's \n\n that?</pre>";
Document doc = Jsoup.parse(h);
assertEquals("Hello there. What's \n\n that?", doc.text());
}
@Test public void testKeepsPreTextInCode() {
String h = "<pre><code>code\n\ncode</code></pre>";
Document doc = Jsoup.parse(h);
assertEquals("code\n\ncode", doc.text());
assertEquals("<pre><code>code\n\ncode</code></pre>", doc.body().html());
}
@Test public void testBrHasSpace() {
Document doc = Jsoup.parse("<p>Hello<br>there</p>");
assertEquals("Hello there", doc.text());
assertEquals("Hello there", doc.select("p").first().ownText());
doc = Jsoup.parse("<p>Hello <br> there</p>");
assertEquals("Hello there", doc.text());
}
@Test public void testGetSiblings() {
Document doc = Jsoup.parse("<div><p>Hello<p id=1>there<p>this<p>is<p>an<p id=last>element</div>");
Element p = doc.getElementById("1");
assertEquals("there", p.text());
assertEquals("Hello", p.previousElementSibling().text());
assertEquals("this", p.nextElementSibling().text());
assertEquals("Hello", p.firstElementSibling().text());
assertEquals("element", p.lastElementSibling().text());
}
@Test public void testGetParents() {
Document doc = Jsoup.parse("<div><p>Hello <span>there</span></div>");
Element span = doc.select("span").first();
Elements parents = span.parents();
assertEquals(4, parents.size());
assertEquals("p", parents.get(0).tagName());
assertEquals("div", parents.get(1).tagName());
assertEquals("body", parents.get(2).tagName());
assertEquals("html", parents.get(3).tagName());
}
@Test public void testElementSiblingIndex() {
Document doc = Jsoup.parse("<div><p>One</p>...<p>Two</p>...<p>Three</p>");
Elements ps = doc.select("p");
assertTrue(0 == ps.get(0).elementSiblingIndex());
assertTrue(1 == ps.get(1).elementSiblingIndex());
assertTrue(2 == ps.get(2).elementSiblingIndex());
}
@Test public void testGetElementsWithClass() {
Document doc = Jsoup.parse("<div class='mellow yellow'><span class=mellow>Hello <b class='yellow'>Yellow!</b></span><p>Empty</p></div>");
List<Element> els = doc.getElementsByClass("mellow");
assertEquals(2, els.size());
assertEquals("div", els.get(0).tagName());
assertEquals("span", els.get(1).tagName());
List<Element> els2 = doc.getElementsByClass("yellow");
assertEquals(2, els2.size());
assertEquals("div", els2.get(0).tagName());
assertEquals("b", els2.get(1).tagName());
List<Element> none = doc.getElementsByClass("solo");
assertEquals(0, none.size());
}
@Test public void testGetElementsWithAttribute() {
Document doc = Jsoup.parse("<div style='bold'><p title=qux><p><b style></b></p></div>");
List<Element> els = doc.getElementsByAttribute("style");
assertEquals(2, els.size());
assertEquals("div", els.get(0).tagName());
assertEquals("b", els.get(1).tagName());
List<Element> none = doc.getElementsByAttribute("class");
assertEquals(0, none.size());
}
@Test public void testGetElementsWithAttributeDash() {
Document doc = Jsoup.parse("<meta http-equiv=content-type value=utf8 id=1> <meta name=foo content=bar id=2> <div http-equiv=content-type value=utf8 id=3>");
Elements meta = doc.select("meta[http-equiv=content-type], meta[charset]");
assertEquals(1, meta.size());
assertEquals("1", meta.first().id());
}
@Test public void testGetElementsWithAttributeValue() {
Document doc = Jsoup.parse("<div style='bold'><p><p><b style></b></p></div>");
List<Element> els = doc.getElementsByAttributeValue("style", "bold");
assertEquals(1, els.size());
assertEquals("div", els.get(0).tagName());
List<Element> none = doc.getElementsByAttributeValue("style", "none");
assertEquals(0, none.size());
}
@Test public void testClassDomMethods() {
Document doc = Jsoup.parse("<div><span class='mellow yellow'>Hello <b>Yellow</b></span></div>");
List<Element> els = doc.getElementsByAttribute("class");
Element span = els.get(0);
assertEquals("mellow yellow", span.className());
assertTrue(span.hasClass("mellow"));
assertTrue(span.hasClass("yellow"));
Set<String> classes = span.classNames();
assertEquals(2, classes.size());
assertTrue(classes.contains("mellow"));
assertTrue(classes.contains("yellow"));
assertEquals("", doc.className());
assertFalse(doc.hasClass("mellow"));
}
@Test public void testClassUpdates() {
Document doc = Jsoup.parse("<div class='mellow yellow'></div>");
Element div = doc.select("div").first();
div.addClass("green");
assertEquals("mellow yellow green", div.className());
div.removeClass("red"); // noop
div.removeClass("yellow");
assertEquals("mellow green", div.className());
div.toggleClass("green").toggleClass("red");
assertEquals("mellow red", div.className());
}
@Test public void testOuterHtml() {
Document doc = Jsoup.parse("<div title='Tags &c.'><img src=foo.png><p><!-- comment -->Hello<p>there");
assertEquals("<html><head></head><body><div title=\"Tags &c.\"><img src=\"foo.png\" /><p><!-- comment -->Hello</p><p>there</p></div></body></html>",
TextUtil.stripNewlines(doc.outerHtml()));
}
@Test public void testInnerHtml() {
Document doc = Jsoup.parse("<div><p>Hello</p></div>");
assertEquals("<p>Hello</p>", doc.getElementsByTag("div").get(0).html());
}
@Test public void testFormatHtml() {
Document doc = Jsoup.parse("<title>Format test</title><div><p>Hello <span>jsoup <span>users</span></span></p><p>Good.</p></div>");
assertEquals("<html>\n <head>\n <title>Format test</title>\n </head>\n <body>\n <div>\n <p>Hello <span>jsoup <span>users</span></span></p>\n <p>Good.</p>\n </div>\n </body>\n</html>", doc.html());
}
@Test public void testSetIndent() {
Document doc = Jsoup.parse("<div><p>Hello\nthere</p></div>");
doc.outputSettings().indentAmount(0);
assertEquals("<html>\n<head></head>\n<body>\n<div>\n<p>Hello there</p>\n</div>\n</body>\n</html>", doc.html());
}
@Test public void testNotPretty() {
Document doc = Jsoup.parse("<div> \n<p>Hello\n there</p></div>");
doc.outputSettings().prettyPrint(false);
assertEquals("<html><head></head><body><div> \n<p>Hello\n there</p></div></body></html>", doc.html());
}
@Test public void testEmptyElementFormatHtml() {
// don't put newlines into empty blocks
Document doc = Jsoup.parse("<section><div></div></section>");
assertEquals("<section>\n <div></div>\n</section>", doc.select("section").first().outerHtml());
}
@Test public void testNoIndentOnScriptAndStyle() {
// don't newline+indent closing </script> and </style> tags
Document doc = Jsoup.parse("<script>one\ntwo</script>\n<style>three\nfour</style>");
assertEquals("<script>one\ntwo</script> \n<style>three\nfour</style>", doc.head().html());
}
@Test public void testContainerOutput() {
Document doc = Jsoup.parse("<title>Hello there</title> <div><p>Hello</p><p>there</p></div> <div>Another</div>");
assertEquals("<title>Hello there</title>", doc.select("title").first().outerHtml());
assertEquals("<div>\n <p>Hello</p>\n <p>there</p>\n</div>", doc.select("div").first().outerHtml());
assertEquals("<div>\n <p>Hello</p>\n <p>there</p>\n</div> \n<div>\n Another\n</div>", doc.select("body").first().html());
}
@Test public void testSetText() {
String h = "<div id=1>Hello <p>there <b>now</b></p></div>";
Document doc = Jsoup.parse(h);
assertEquals("Hello there now", doc.text()); // need to sort out node whitespace
assertEquals("there now", doc.select("p").get(0).text());
Element div = doc.getElementById("1").text("Gone");
assertEquals("Gone", div.text());
assertEquals(0, doc.select("p").size());
}
@Test public void testAddNewElement() {
Document doc = Jsoup.parse("<div id=1><p>Hello</p></div>");
Element div = doc.getElementById("1");
div.appendElement("p").text("there");
div.appendElement("P").attr("class", "second").text("now");
assertEquals("<html><head></head><body><div id=\"1\"><p>Hello</p><p>there</p><p class=\"second\">now</p></div></body></html>",
TextUtil.stripNewlines(doc.html()));
// check sibling index (with short circuit on reindexChildren):
Elements ps = doc.select("p");
for (int i = 0; i < ps.size(); i++) {
assertEquals(i, ps.get(i).siblingIndex);
}
}
@Test public void testAppendRowToTable() {
Document doc = Jsoup.parse("<table><tr><td>1</td></tr></table>");
Element table = doc.select("tbody").first();
table.append("<tr><td>2</td></tr>");
assertEquals("<table><tbody><tr><td>1</td></tr><tr><td>2</td></tr></tbody></table>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void testPrependRowToTable() {
Document doc = Jsoup.parse("<table><tr><td>1</td></tr></table>");
Element table = doc.select("tbody").first();
table.prepend("<tr><td>2</td></tr>");
assertEquals("<table><tbody><tr><td>2</td></tr><tr><td>1</td></tr></tbody></table>", TextUtil.stripNewlines(doc.body().html()));
// check sibling index (reindexChildren):
Elements ps = doc.select("tr");
for (int i = 0; i < ps.size(); i++) {
assertEquals(i, ps.get(i).siblingIndex);
}
}
@Test public void testPrependElement() {
Document doc = Jsoup.parse("<div id=1><p>Hello</p></div>");
Element div = doc.getElementById("1");
div.prependElement("p").text("Before");
assertEquals("Before", div.child(0).text());
assertEquals("Hello", div.child(1).text());
}
@Test public void testAddNewText() {
Document doc = Jsoup.parse("<div id=1><p>Hello</p></div>");
Element div = doc.getElementById("1");
div.appendText(" there & now >");
assertEquals("<p>Hello</p> there & now >", TextUtil.stripNewlines(div.html()));
}
@Test public void testPrependText() {
Document doc = Jsoup.parse("<div id=1><p>Hello</p></div>");
Element div = doc.getElementById("1");
div.prependText("there & now > ");
assertEquals("there & now > Hello", div.text());
assertEquals("there & now > <p>Hello</p>", TextUtil.stripNewlines(div.html()));
}
@Test public void testAddNewHtml() {
Document doc = Jsoup.parse("<div id=1><p>Hello</p></div>");
Element div = doc.getElementById("1");
div.append("<p>there</p><p>now</p>");
assertEquals("<p>Hello</p><p>there</p><p>now</p>", TextUtil.stripNewlines(div.html()));
// check sibling index (no reindexChildren):
Elements ps = doc.select("p");
for (int i = 0; i < ps.size(); i++) {
assertEquals(i, ps.get(i).siblingIndex);
}
}
@Test public void testPrependNewHtml() {
Document doc = Jsoup.parse("<div id=1><p>Hello</p></div>");
Element div = doc.getElementById("1");
div.prepend("<p>there</p><p>now</p>");
assertEquals("<p>there</p><p>now</p><p>Hello</p>", TextUtil.stripNewlines(div.html()));
// check sibling index (reindexChildren):
Elements ps = doc.select("p");
for (int i = 0; i < ps.size(); i++) {
assertEquals(i, ps.get(i).siblingIndex);
}
}
@Test public void testSetHtml() {
Document doc = Jsoup.parse("<div id=1><p>Hello</p></div>");
Element div = doc.getElementById("1");
div.html("<p>there</p><p>now</p>");
assertEquals("<p>there</p><p>now</p>", TextUtil.stripNewlines(div.html()));
}
@Test public void testWrap() {
Document doc = Jsoup.parse("<div><p>Hello</p><p>There</p></div>");
Element p = doc.select("p").first();
p.wrap("<div class='head'></div>");
assertEquals("<div><div class=\"head\"><p>Hello</p></div><p>There</p></div>", TextUtil.stripNewlines(doc.body().html()));
Element ret = p.wrap("<div><div class=foo></div><p>What?</p></div>");
assertEquals("<div><div class=\"head\"><div><div class=\"foo\"><p>Hello</p></div><p>What?</p></div></div><p>There</p></div>",
TextUtil.stripNewlines(doc.body().html()));
assertEquals(ret, p);
}
@Test public void before() {
Document doc = Jsoup.parse("<div><p>Hello</p><p>There</p></div>");
Element p1 = doc.select("p").first();
p1.before("<div>one</div><div>two</div>");
assertEquals("<div><div>one</div><div>two</div><p>Hello</p><p>There</p></div>", TextUtil.stripNewlines(doc.body().html()));
doc.select("p").last().before("<p>Three</p><!-- four -->");
assertEquals("<div><div>one</div><div>two</div><p>Hello</p><p>Three</p><!-- four --><p>There</p></div>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void after() {
Document doc = Jsoup.parse("<div><p>Hello</p><p>There</p></div>");
Element p1 = doc.select("p").first();
p1.after("<div>one</div><div>two</div>");
assertEquals("<div><p>Hello</p><div>one</div><div>two</div><p>There</p></div>", TextUtil.stripNewlines(doc.body().html()));
doc.select("p").last().after("<p>Three</p><!-- four -->");
assertEquals("<div><p>Hello</p><div>one</div><div>two</div><p>There</p><p>Three</p><!-- four --></div>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void testWrapWithRemainder() {
Document doc = Jsoup.parse("<div><p>Hello</p></div>");
Element p = doc.select("p").first();
p.wrap("<div class='head'></div><p>There!</p>");
assertEquals("<div><div class=\"head\"><p>Hello</p><p>There!</p></div></div>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void testHasText() {
Document doc = Jsoup.parse("<div><p>Hello</p><p></p></div>");
Element div = doc.select("div").first();
Elements ps = doc.select("p");
assertTrue(div.hasText());
assertTrue(ps.first().hasText());
assertFalse(ps.last().hasText());
}
@Test public void dataset() {
Document doc = Jsoup.parse("<div id=1 data-name=jsoup class=new data-package=jar>Hello</div><p id=2>Hello</p>");
Element div = doc.select("div").first();
Map<String, String> dataset = div.dataset();
Attributes attributes = div.attributes();
// size, get, set, add, remove
assertEquals(2, dataset.size());
assertEquals("jsoup", dataset.get("name"));
assertEquals("jar", dataset.get("package"));
dataset.put("name", "jsoup updated");
dataset.put("language", "java");
dataset.remove("package");
assertEquals(2, dataset.size());
assertEquals(4, attributes.size());
assertEquals("jsoup updated", attributes.get("data-name"));
assertEquals("jsoup updated", dataset.get("name"));
assertEquals("java", attributes.get("data-language"));
assertEquals("java", dataset.get("language"));
attributes.put("data-food", "bacon");
assertEquals(3, dataset.size());
assertEquals("bacon", dataset.get("food"));
attributes.put("data-", "empty");
assertEquals(null, dataset.get("")); // data- is not a data attribute
Element p = doc.select("p").first();
assertEquals(0, p.dataset().size());
}
@Test public void parentlessToString() {
Document doc = Jsoup.parse("<img src='foo'>");
Element img = doc.select("img").first();
assertEquals("<img src=\"foo\" />", img.toString());
img.remove(); // lost its parent
assertEquals("<img src=\"foo\" />", img.toString());
}
@Test public void testClone() {
Document doc = Jsoup.parse("<div><p>One<p><span>Two</div>");
Element p = doc.select("p").get(1);
Element clone = p.clone();
assertNull(clone.parent()); // should be orphaned
assertEquals(0, clone.siblingIndex);
assertEquals(1, p.siblingIndex);
assertNotNull(p.parent());
clone.append("<span>Three");
assertEquals("<p><span>Two</span><span>Three</span></p>", TextUtil.stripNewlines(clone.outerHtml()));
assertEquals("<div><p>One</p><p><span>Two</span></p></div>", TextUtil.stripNewlines(doc.body().html())); // not modified
doc.body().appendChild(clone); // adopt
assertNotNull(clone.parent());
assertEquals("<div><p>One</p><p><span>Two</span></p></div><p><span>Two</span><span>Three</span></p>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void testClonesClassnames() {
Document doc = Jsoup.parse("<div class='one two'></div>");
Element div = doc.select("div").first();
Set<String> classes = div.classNames();
assertEquals(2, classes.size());
assertTrue(classes.contains("one"));
assertTrue(classes.contains("two"));
Element copy = div.clone();
Set<String> copyClasses = copy.classNames();
assertEquals(2, copyClasses.size());
assertTrue(copyClasses.contains("one"));
assertTrue(copyClasses.contains("two"));
copyClasses.add("three");
copyClasses.remove("one");
assertTrue(classes.contains("one"));
assertFalse(classes.contains("three"));
assertFalse(copyClasses.contains("one"));
assertTrue(copyClasses.contains("three"));
assertEquals("", div.html());
assertEquals("", copy.html());
}
@Test public void testTagNameSet() {
Document doc = Jsoup.parse("<div><i>Hello</i>");
doc.select("i").first().tagName("em");
assertEquals(0, doc.select("i").size());
assertEquals(1, doc.select("em").size());
assertEquals("<em>Hello</em>", doc.select("div").first().html());
}
@Test public void testHtmlContainsOuter() {
Document doc = Jsoup.parse("<title>Check</title> <div>Hello there</div>");
doc.outputSettings().indentAmount(0);
assertTrue(doc.html().contains(doc.select("title").outerHtml()));
assertTrue(doc.html().contains(doc.select("div").outerHtml()));
}
@Test public void testGetTextNodes() {
Document doc = Jsoup.parse("<p>One <span>Two</span> Three <br> Four</p>");
List<TextNode> textNodes = doc.select("p").first().textNodes();
assertEquals(3, textNodes.size());
assertEquals("One ", textNodes.get(0).text());
assertEquals(" Three ", textNodes.get(1).text());
assertEquals(" Four", textNodes.get(2).text());
assertEquals(0, doc.select("br").first().textNodes().size());
}
@Test public void testManipulateTextNodes() {
Document doc = Jsoup.parse("<p>One <span>Two</span> Three <br> Four</p>");
Element p = doc.select("p").first();
List<TextNode> textNodes = p.textNodes();
textNodes.get(1).text(" three-more ");
textNodes.get(2).splitText(3).text("-ur");
assertEquals("One Two three-more Fo-ur", p.text());
assertEquals("One three-more Fo-ur", p.ownText());
assertEquals(4, p.textNodes().size()); // grew because of split
}
@Test public void testGetDataNodes() {
Document doc = Jsoup.parse("<script>One Two</script> <style>Three Four</style> <p>Fix Six</p>");
Element script = doc.select("script").first();
Element style = doc.select("style").first();
Element p = doc.select("p").first();
List<DataNode> scriptData = script.dataNodes();
assertEquals(1, scriptData.size());
assertEquals("One Two", scriptData.get(0).getWholeData());
List<DataNode> styleData = style.dataNodes();
assertEquals(1, styleData.size());
assertEquals("Three Four", styleData.get(0).getWholeData());
List<DataNode> pData = p.dataNodes();
assertEquals(0, pData.size());
}
@Test public void elementIsNotASiblingOfItself() {
Document doc = Jsoup.parse("<div><p>One<p>Two<p>Three</div>");
Element p2 = doc.select("p").get(1);
assertEquals("Two", p2.text());
Elements els = p2.siblingElements();
assertEquals(2, els.size());
assertEquals("<p>One</p>", els.get(0).outerHtml());
assertEquals("<p>Three</p>", els.get(1).outerHtml());
}
@Test public void testChildThrowsIndexOutOfBoundsOnMissing() {
Document doc = Jsoup.parse("<div><p>One</p><p>Two</p></div>");
Element div = doc.select("div").first();
assertEquals(2, div.children().size());
assertEquals("One", div.child(0).text());
try {
div.child(3);
fail("Should throw index out of bounds");
} catch (IndexOutOfBoundsException e) {}
}
@Test
public void moveByAppend() {
// test for https://github.com/jhy/jsoup/issues/239
// can empty an element and append its children to another element
Document doc = Jsoup.parse("<div id=1>Text <p>One</p> Text <p>Two</p></div><div id=2></div>");
Element div1 = doc.select("div").get(0);
Element div2 = doc.select("div").get(1);
assertEquals(4, div1.childNodeSize());
List<Node> children = div1.childNodes();
assertEquals(4, children.size());
div2.insertChildren(0, children);
assertEquals(0, children.size()); // children is backed by div1.childNodes, moved, so should be 0 now
assertEquals(0, div1.childNodeSize());
assertEquals(4, div2.childNodeSize());
assertEquals("<div id=\"1\"></div>\n<div id=\"2\">\n Text \n <p>One</p> Text \n <p>Two</p>\n</div>",
doc.body().html());
}
@Test
public void insertChildrenArgumentValidation() {
Document doc = Jsoup.parse("<div id=1>Text <p>One</p> Text <p>Two</p></div><div id=2></div>");
Element div1 = doc.select("div").get(0);
Element div2 = doc.select("div").get(1);
List<Node> children = div1.childNodes();
try {
div2.insertChildren(6, children);
fail();
} catch (IllegalArgumentException e) {}
try {
div2.insertChildren(-5, children);
fail();
} catch (IllegalArgumentException e) {
}
try {
div2.insertChildren(0, null);
fail();
} catch (IllegalArgumentException e) {
}
}
@Test
public void insertChildrenAtPosition() {
Document doc = Jsoup.parse("<div id=1>Text1 <p>One</p> Text2 <p>Two</p></div><div id=2>Text3 <p>Three</p></div>");
Element div1 = doc.select("div").get(0);
Elements p1s = div1.select("p");
Element div2 = doc.select("div").get(1);
assertEquals(2, div2.childNodeSize());
div2.insertChildren(-1, p1s);
assertEquals(2, div1.childNodeSize()); // moved two out
assertEquals(4, div2.childNodeSize());
assertEquals(3, p1s.get(1).siblingIndex()); // should be last
List<Node> els = new ArrayList<Node>();
Element el1 = new Element(Tag.valueOf("span"), "").text("Span1");
Element el2 = new Element(Tag.valueOf("span"), "").text("Span2");
TextNode tn1 = new TextNode("Text4", "");
els.add(el1);
els.add(el2);
els.add(tn1);
assertNull(el1.parent());
div2.insertChildren(-2, els);
assertEquals(div2, el1.parent());
assertEquals(7, div2.childNodeSize());
assertEquals(3, el1.siblingIndex());
assertEquals(4, el2.siblingIndex());
assertEquals(5, tn1.siblingIndex());
}
@Test
public void insertChildrenAsCopy() {
Document doc = Jsoup.parse("<div id=1>Text <p>One</p> Text <p>Two</p></div><div id=2></div>");
Element div1 = doc.select("div").get(0);
Element div2 = doc.select("div").get(1);
Elements ps = doc.select("p").clone();
ps.first().text("One cloned");
div2.insertChildren(-1, ps);
assertEquals(4, div1.childNodeSize()); // not moved -- cloned
assertEquals(2, div2.childNodeSize());
assertEquals("<div id=\"1\">Text <p>One</p> Text <p>Two</p></div><div id=\"2\"><p>One cloned</p><p>Two</p></div>",
TextUtil.stripNewlines(doc.body().html()));
}
} | // You are a professional Java test case writer, please create a test case named `testClonesClassnames` for the issue `Jsoup-278`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Jsoup-278
//
// ## Issue-Title:
// Element.clone() wrongly shared a same classNames Set instance
//
// ## Issue-Description:
// In the clone() method of Node, the Object.clone() is called, if the original element's classNames Set had been initialized before clone, the original classNames Set will be set to the new cloned Element instance due to the JDK's clone mechanism. Thus, the old element and the newly cloned Element will share a same classNames Set instance.
//
//
//
//
@Test public void testClonesClassnames() {
| 499 | 32 | 476 | src/test/java/org/jsoup/nodes/ElementTest.java | src/test/java | ```markdown
## Issue-ID: Jsoup-278
## Issue-Title:
Element.clone() wrongly shared a same classNames Set instance
## Issue-Description:
In the clone() method of Node, the Object.clone() is called, if the original element's classNames Set had been initialized before clone, the original classNames Set will be set to the new cloned Element instance due to the JDK's clone mechanism. Thus, the old element and the newly cloned Element will share a same classNames Set instance.
```
You are a professional Java test case writer, please create a test case named `testClonesClassnames` for the issue `Jsoup-278`, utilizing the provided issue report information and the following function signature.
```java
@Test public void testClonesClassnames() {
```
| 476 | [
"org.jsoup.nodes.Element"
] | 7ce1e39f3ff949f2ae90a29e858145e371f14ce0782d3981c0104c89d6b50587 | @Test public void testClonesClassnames() | // You are a professional Java test case writer, please create a test case named `testClonesClassnames` for the issue `Jsoup-278`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Jsoup-278
//
// ## Issue-Title:
// Element.clone() wrongly shared a same classNames Set instance
//
// ## Issue-Description:
// In the clone() method of Node, the Object.clone() is called, if the original element's classNames Set had been initialized before clone, the original classNames Set will be set to the new cloned Element instance due to the JDK's clone mechanism. Thus, the old element and the newly cloned Element will share a same classNames Set instance.
//
//
//
//
| Jsoup | package org.jsoup.nodes;
import org.jsoup.Jsoup;
import org.jsoup.TextUtil;
import org.jsoup.helper.StringUtil;
import org.jsoup.parser.Tag;
import org.jsoup.select.Elements;
import org.junit.Test;
import static org.junit.Assert.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Set;
import java.util.Map;
/**
* Tests for Element (DOM stuff mostly).
*
* @author Jonathan Hedley
*/
public class ElementTest {
private String reference = "<div id=div1><p>Hello</p><p>Another <b>element</b></p><div id=div2><img src=foo.png></div></div>";
@Test public void getElementsByTagName() {
Document doc = Jsoup.parse(reference);
List<Element> divs = doc.getElementsByTag("div");
assertEquals(2, divs.size());
assertEquals("div1", divs.get(0).id());
assertEquals("div2", divs.get(1).id());
List<Element> ps = doc.getElementsByTag("p");
assertEquals(2, ps.size());
assertEquals("Hello", ((TextNode) ps.get(0).childNode(0)).getWholeText());
assertEquals("Another ", ((TextNode) ps.get(1).childNode(0)).getWholeText());
List<Element> ps2 = doc.getElementsByTag("P");
assertEquals(ps, ps2);
List<Element> imgs = doc.getElementsByTag("img");
assertEquals("foo.png", imgs.get(0).attr("src"));
List<Element> empty = doc.getElementsByTag("wtf");
assertEquals(0, empty.size());
}
@Test public void getNamespacedElementsByTag() {
Document doc = Jsoup.parse("<div><abc:def id=1>Hello</abc:def></div>");
Elements els = doc.getElementsByTag("abc:def");
assertEquals(1, els.size());
assertEquals("1", els.first().id());
assertEquals("abc:def", els.first().tagName());
}
@Test public void testGetElementById() {
Document doc = Jsoup.parse(reference);
Element div = doc.getElementById("div1");
assertEquals("div1", div.id());
assertNull(doc.getElementById("none"));
Document doc2 = Jsoup.parse("<div id=1><div id=2><p>Hello <span id=2>world!</span></p></div></div>");
Element div2 = doc2.getElementById("2");
assertEquals("div", div2.tagName()); // not the span
Element span = div2.child(0).getElementById("2"); // called from <p> context should be span
assertEquals("span", span.tagName());
}
@Test public void testGetText() {
Document doc = Jsoup.parse(reference);
assertEquals("Hello Another element", doc.text());
assertEquals("Another element", doc.getElementsByTag("p").get(1).text());
}
@Test public void testGetChildText() {
Document doc = Jsoup.parse("<p>Hello <b>there</b> now");
Element p = doc.select("p").first();
assertEquals("Hello there now", p.text());
assertEquals("Hello now", p.ownText());
}
@Test public void testNormalisesText() {
String h = "<p>Hello<p>There.</p> \n <p>Here <b>is</b> \n s<b>om</b>e text.";
Document doc = Jsoup.parse(h);
String text = doc.text();
assertEquals("Hello There. Here is some text.", text);
}
@Test public void testKeepsPreText() {
String h = "<p>Hello \n \n there.</p> <div><pre> What's \n\n that?</pre>";
Document doc = Jsoup.parse(h);
assertEquals("Hello there. What's \n\n that?", doc.text());
}
@Test public void testKeepsPreTextInCode() {
String h = "<pre><code>code\n\ncode</code></pre>";
Document doc = Jsoup.parse(h);
assertEquals("code\n\ncode", doc.text());
assertEquals("<pre><code>code\n\ncode</code></pre>", doc.body().html());
}
@Test public void testBrHasSpace() {
Document doc = Jsoup.parse("<p>Hello<br>there</p>");
assertEquals("Hello there", doc.text());
assertEquals("Hello there", doc.select("p").first().ownText());
doc = Jsoup.parse("<p>Hello <br> there</p>");
assertEquals("Hello there", doc.text());
}
@Test public void testGetSiblings() {
Document doc = Jsoup.parse("<div><p>Hello<p id=1>there<p>this<p>is<p>an<p id=last>element</div>");
Element p = doc.getElementById("1");
assertEquals("there", p.text());
assertEquals("Hello", p.previousElementSibling().text());
assertEquals("this", p.nextElementSibling().text());
assertEquals("Hello", p.firstElementSibling().text());
assertEquals("element", p.lastElementSibling().text());
}
@Test public void testGetParents() {
Document doc = Jsoup.parse("<div><p>Hello <span>there</span></div>");
Element span = doc.select("span").first();
Elements parents = span.parents();
assertEquals(4, parents.size());
assertEquals("p", parents.get(0).tagName());
assertEquals("div", parents.get(1).tagName());
assertEquals("body", parents.get(2).tagName());
assertEquals("html", parents.get(3).tagName());
}
@Test public void testElementSiblingIndex() {
Document doc = Jsoup.parse("<div><p>One</p>...<p>Two</p>...<p>Three</p>");
Elements ps = doc.select("p");
assertTrue(0 == ps.get(0).elementSiblingIndex());
assertTrue(1 == ps.get(1).elementSiblingIndex());
assertTrue(2 == ps.get(2).elementSiblingIndex());
}
@Test public void testGetElementsWithClass() {
Document doc = Jsoup.parse("<div class='mellow yellow'><span class=mellow>Hello <b class='yellow'>Yellow!</b></span><p>Empty</p></div>");
List<Element> els = doc.getElementsByClass("mellow");
assertEquals(2, els.size());
assertEquals("div", els.get(0).tagName());
assertEquals("span", els.get(1).tagName());
List<Element> els2 = doc.getElementsByClass("yellow");
assertEquals(2, els2.size());
assertEquals("div", els2.get(0).tagName());
assertEquals("b", els2.get(1).tagName());
List<Element> none = doc.getElementsByClass("solo");
assertEquals(0, none.size());
}
@Test public void testGetElementsWithAttribute() {
Document doc = Jsoup.parse("<div style='bold'><p title=qux><p><b style></b></p></div>");
List<Element> els = doc.getElementsByAttribute("style");
assertEquals(2, els.size());
assertEquals("div", els.get(0).tagName());
assertEquals("b", els.get(1).tagName());
List<Element> none = doc.getElementsByAttribute("class");
assertEquals(0, none.size());
}
@Test public void testGetElementsWithAttributeDash() {
Document doc = Jsoup.parse("<meta http-equiv=content-type value=utf8 id=1> <meta name=foo content=bar id=2> <div http-equiv=content-type value=utf8 id=3>");
Elements meta = doc.select("meta[http-equiv=content-type], meta[charset]");
assertEquals(1, meta.size());
assertEquals("1", meta.first().id());
}
@Test public void testGetElementsWithAttributeValue() {
Document doc = Jsoup.parse("<div style='bold'><p><p><b style></b></p></div>");
List<Element> els = doc.getElementsByAttributeValue("style", "bold");
assertEquals(1, els.size());
assertEquals("div", els.get(0).tagName());
List<Element> none = doc.getElementsByAttributeValue("style", "none");
assertEquals(0, none.size());
}
@Test public void testClassDomMethods() {
Document doc = Jsoup.parse("<div><span class='mellow yellow'>Hello <b>Yellow</b></span></div>");
List<Element> els = doc.getElementsByAttribute("class");
Element span = els.get(0);
assertEquals("mellow yellow", span.className());
assertTrue(span.hasClass("mellow"));
assertTrue(span.hasClass("yellow"));
Set<String> classes = span.classNames();
assertEquals(2, classes.size());
assertTrue(classes.contains("mellow"));
assertTrue(classes.contains("yellow"));
assertEquals("", doc.className());
assertFalse(doc.hasClass("mellow"));
}
@Test public void testClassUpdates() {
Document doc = Jsoup.parse("<div class='mellow yellow'></div>");
Element div = doc.select("div").first();
div.addClass("green");
assertEquals("mellow yellow green", div.className());
div.removeClass("red"); // noop
div.removeClass("yellow");
assertEquals("mellow green", div.className());
div.toggleClass("green").toggleClass("red");
assertEquals("mellow red", div.className());
}
@Test public void testOuterHtml() {
Document doc = Jsoup.parse("<div title='Tags &c.'><img src=foo.png><p><!-- comment -->Hello<p>there");
assertEquals("<html><head></head><body><div title=\"Tags &c.\"><img src=\"foo.png\" /><p><!-- comment -->Hello</p><p>there</p></div></body></html>",
TextUtil.stripNewlines(doc.outerHtml()));
}
@Test public void testInnerHtml() {
Document doc = Jsoup.parse("<div><p>Hello</p></div>");
assertEquals("<p>Hello</p>", doc.getElementsByTag("div").get(0).html());
}
@Test public void testFormatHtml() {
Document doc = Jsoup.parse("<title>Format test</title><div><p>Hello <span>jsoup <span>users</span></span></p><p>Good.</p></div>");
assertEquals("<html>\n <head>\n <title>Format test</title>\n </head>\n <body>\n <div>\n <p>Hello <span>jsoup <span>users</span></span></p>\n <p>Good.</p>\n </div>\n </body>\n</html>", doc.html());
}
@Test public void testSetIndent() {
Document doc = Jsoup.parse("<div><p>Hello\nthere</p></div>");
doc.outputSettings().indentAmount(0);
assertEquals("<html>\n<head></head>\n<body>\n<div>\n<p>Hello there</p>\n</div>\n</body>\n</html>", doc.html());
}
@Test public void testNotPretty() {
Document doc = Jsoup.parse("<div> \n<p>Hello\n there</p></div>");
doc.outputSettings().prettyPrint(false);
assertEquals("<html><head></head><body><div> \n<p>Hello\n there</p></div></body></html>", doc.html());
}
@Test public void testEmptyElementFormatHtml() {
// don't put newlines into empty blocks
Document doc = Jsoup.parse("<section><div></div></section>");
assertEquals("<section>\n <div></div>\n</section>", doc.select("section").first().outerHtml());
}
@Test public void testNoIndentOnScriptAndStyle() {
// don't newline+indent closing </script> and </style> tags
Document doc = Jsoup.parse("<script>one\ntwo</script>\n<style>three\nfour</style>");
assertEquals("<script>one\ntwo</script> \n<style>three\nfour</style>", doc.head().html());
}
@Test public void testContainerOutput() {
Document doc = Jsoup.parse("<title>Hello there</title> <div><p>Hello</p><p>there</p></div> <div>Another</div>");
assertEquals("<title>Hello there</title>", doc.select("title").first().outerHtml());
assertEquals("<div>\n <p>Hello</p>\n <p>there</p>\n</div>", doc.select("div").first().outerHtml());
assertEquals("<div>\n <p>Hello</p>\n <p>there</p>\n</div> \n<div>\n Another\n</div>", doc.select("body").first().html());
}
@Test public void testSetText() {
String h = "<div id=1>Hello <p>there <b>now</b></p></div>";
Document doc = Jsoup.parse(h);
assertEquals("Hello there now", doc.text()); // need to sort out node whitespace
assertEquals("there now", doc.select("p").get(0).text());
Element div = doc.getElementById("1").text("Gone");
assertEquals("Gone", div.text());
assertEquals(0, doc.select("p").size());
}
@Test public void testAddNewElement() {
Document doc = Jsoup.parse("<div id=1><p>Hello</p></div>");
Element div = doc.getElementById("1");
div.appendElement("p").text("there");
div.appendElement("P").attr("class", "second").text("now");
assertEquals("<html><head></head><body><div id=\"1\"><p>Hello</p><p>there</p><p class=\"second\">now</p></div></body></html>",
TextUtil.stripNewlines(doc.html()));
// check sibling index (with short circuit on reindexChildren):
Elements ps = doc.select("p");
for (int i = 0; i < ps.size(); i++) {
assertEquals(i, ps.get(i).siblingIndex);
}
}
@Test public void testAppendRowToTable() {
Document doc = Jsoup.parse("<table><tr><td>1</td></tr></table>");
Element table = doc.select("tbody").first();
table.append("<tr><td>2</td></tr>");
assertEquals("<table><tbody><tr><td>1</td></tr><tr><td>2</td></tr></tbody></table>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void testPrependRowToTable() {
Document doc = Jsoup.parse("<table><tr><td>1</td></tr></table>");
Element table = doc.select("tbody").first();
table.prepend("<tr><td>2</td></tr>");
assertEquals("<table><tbody><tr><td>2</td></tr><tr><td>1</td></tr></tbody></table>", TextUtil.stripNewlines(doc.body().html()));
// check sibling index (reindexChildren):
Elements ps = doc.select("tr");
for (int i = 0; i < ps.size(); i++) {
assertEquals(i, ps.get(i).siblingIndex);
}
}
@Test public void testPrependElement() {
Document doc = Jsoup.parse("<div id=1><p>Hello</p></div>");
Element div = doc.getElementById("1");
div.prependElement("p").text("Before");
assertEquals("Before", div.child(0).text());
assertEquals("Hello", div.child(1).text());
}
@Test public void testAddNewText() {
Document doc = Jsoup.parse("<div id=1><p>Hello</p></div>");
Element div = doc.getElementById("1");
div.appendText(" there & now >");
assertEquals("<p>Hello</p> there & now >", TextUtil.stripNewlines(div.html()));
}
@Test public void testPrependText() {
Document doc = Jsoup.parse("<div id=1><p>Hello</p></div>");
Element div = doc.getElementById("1");
div.prependText("there & now > ");
assertEquals("there & now > Hello", div.text());
assertEquals("there & now > <p>Hello</p>", TextUtil.stripNewlines(div.html()));
}
@Test public void testAddNewHtml() {
Document doc = Jsoup.parse("<div id=1><p>Hello</p></div>");
Element div = doc.getElementById("1");
div.append("<p>there</p><p>now</p>");
assertEquals("<p>Hello</p><p>there</p><p>now</p>", TextUtil.stripNewlines(div.html()));
// check sibling index (no reindexChildren):
Elements ps = doc.select("p");
for (int i = 0; i < ps.size(); i++) {
assertEquals(i, ps.get(i).siblingIndex);
}
}
@Test public void testPrependNewHtml() {
Document doc = Jsoup.parse("<div id=1><p>Hello</p></div>");
Element div = doc.getElementById("1");
div.prepend("<p>there</p><p>now</p>");
assertEquals("<p>there</p><p>now</p><p>Hello</p>", TextUtil.stripNewlines(div.html()));
// check sibling index (reindexChildren):
Elements ps = doc.select("p");
for (int i = 0; i < ps.size(); i++) {
assertEquals(i, ps.get(i).siblingIndex);
}
}
@Test public void testSetHtml() {
Document doc = Jsoup.parse("<div id=1><p>Hello</p></div>");
Element div = doc.getElementById("1");
div.html("<p>there</p><p>now</p>");
assertEquals("<p>there</p><p>now</p>", TextUtil.stripNewlines(div.html()));
}
@Test public void testWrap() {
Document doc = Jsoup.parse("<div><p>Hello</p><p>There</p></div>");
Element p = doc.select("p").first();
p.wrap("<div class='head'></div>");
assertEquals("<div><div class=\"head\"><p>Hello</p></div><p>There</p></div>", TextUtil.stripNewlines(doc.body().html()));
Element ret = p.wrap("<div><div class=foo></div><p>What?</p></div>");
assertEquals("<div><div class=\"head\"><div><div class=\"foo\"><p>Hello</p></div><p>What?</p></div></div><p>There</p></div>",
TextUtil.stripNewlines(doc.body().html()));
assertEquals(ret, p);
}
@Test public void before() {
Document doc = Jsoup.parse("<div><p>Hello</p><p>There</p></div>");
Element p1 = doc.select("p").first();
p1.before("<div>one</div><div>two</div>");
assertEquals("<div><div>one</div><div>two</div><p>Hello</p><p>There</p></div>", TextUtil.stripNewlines(doc.body().html()));
doc.select("p").last().before("<p>Three</p><!-- four -->");
assertEquals("<div><div>one</div><div>two</div><p>Hello</p><p>Three</p><!-- four --><p>There</p></div>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void after() {
Document doc = Jsoup.parse("<div><p>Hello</p><p>There</p></div>");
Element p1 = doc.select("p").first();
p1.after("<div>one</div><div>two</div>");
assertEquals("<div><p>Hello</p><div>one</div><div>two</div><p>There</p></div>", TextUtil.stripNewlines(doc.body().html()));
doc.select("p").last().after("<p>Three</p><!-- four -->");
assertEquals("<div><p>Hello</p><div>one</div><div>two</div><p>There</p><p>Three</p><!-- four --></div>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void testWrapWithRemainder() {
Document doc = Jsoup.parse("<div><p>Hello</p></div>");
Element p = doc.select("p").first();
p.wrap("<div class='head'></div><p>There!</p>");
assertEquals("<div><div class=\"head\"><p>Hello</p><p>There!</p></div></div>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void testHasText() {
Document doc = Jsoup.parse("<div><p>Hello</p><p></p></div>");
Element div = doc.select("div").first();
Elements ps = doc.select("p");
assertTrue(div.hasText());
assertTrue(ps.first().hasText());
assertFalse(ps.last().hasText());
}
@Test public void dataset() {
Document doc = Jsoup.parse("<div id=1 data-name=jsoup class=new data-package=jar>Hello</div><p id=2>Hello</p>");
Element div = doc.select("div").first();
Map<String, String> dataset = div.dataset();
Attributes attributes = div.attributes();
// size, get, set, add, remove
assertEquals(2, dataset.size());
assertEquals("jsoup", dataset.get("name"));
assertEquals("jar", dataset.get("package"));
dataset.put("name", "jsoup updated");
dataset.put("language", "java");
dataset.remove("package");
assertEquals(2, dataset.size());
assertEquals(4, attributes.size());
assertEquals("jsoup updated", attributes.get("data-name"));
assertEquals("jsoup updated", dataset.get("name"));
assertEquals("java", attributes.get("data-language"));
assertEquals("java", dataset.get("language"));
attributes.put("data-food", "bacon");
assertEquals(3, dataset.size());
assertEquals("bacon", dataset.get("food"));
attributes.put("data-", "empty");
assertEquals(null, dataset.get("")); // data- is not a data attribute
Element p = doc.select("p").first();
assertEquals(0, p.dataset().size());
}
@Test public void parentlessToString() {
Document doc = Jsoup.parse("<img src='foo'>");
Element img = doc.select("img").first();
assertEquals("<img src=\"foo\" />", img.toString());
img.remove(); // lost its parent
assertEquals("<img src=\"foo\" />", img.toString());
}
@Test public void testClone() {
Document doc = Jsoup.parse("<div><p>One<p><span>Two</div>");
Element p = doc.select("p").get(1);
Element clone = p.clone();
assertNull(clone.parent()); // should be orphaned
assertEquals(0, clone.siblingIndex);
assertEquals(1, p.siblingIndex);
assertNotNull(p.parent());
clone.append("<span>Three");
assertEquals("<p><span>Two</span><span>Three</span></p>", TextUtil.stripNewlines(clone.outerHtml()));
assertEquals("<div><p>One</p><p><span>Two</span></p></div>", TextUtil.stripNewlines(doc.body().html())); // not modified
doc.body().appendChild(clone); // adopt
assertNotNull(clone.parent());
assertEquals("<div><p>One</p><p><span>Two</span></p></div><p><span>Two</span><span>Three</span></p>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void testClonesClassnames() {
Document doc = Jsoup.parse("<div class='one two'></div>");
Element div = doc.select("div").first();
Set<String> classes = div.classNames();
assertEquals(2, classes.size());
assertTrue(classes.contains("one"));
assertTrue(classes.contains("two"));
Element copy = div.clone();
Set<String> copyClasses = copy.classNames();
assertEquals(2, copyClasses.size());
assertTrue(copyClasses.contains("one"));
assertTrue(copyClasses.contains("two"));
copyClasses.add("three");
copyClasses.remove("one");
assertTrue(classes.contains("one"));
assertFalse(classes.contains("three"));
assertFalse(copyClasses.contains("one"));
assertTrue(copyClasses.contains("three"));
assertEquals("", div.html());
assertEquals("", copy.html());
}
@Test public void testTagNameSet() {
Document doc = Jsoup.parse("<div><i>Hello</i>");
doc.select("i").first().tagName("em");
assertEquals(0, doc.select("i").size());
assertEquals(1, doc.select("em").size());
assertEquals("<em>Hello</em>", doc.select("div").first().html());
}
@Test public void testHtmlContainsOuter() {
Document doc = Jsoup.parse("<title>Check</title> <div>Hello there</div>");
doc.outputSettings().indentAmount(0);
assertTrue(doc.html().contains(doc.select("title").outerHtml()));
assertTrue(doc.html().contains(doc.select("div").outerHtml()));
}
@Test public void testGetTextNodes() {
Document doc = Jsoup.parse("<p>One <span>Two</span> Three <br> Four</p>");
List<TextNode> textNodes = doc.select("p").first().textNodes();
assertEquals(3, textNodes.size());
assertEquals("One ", textNodes.get(0).text());
assertEquals(" Three ", textNodes.get(1).text());
assertEquals(" Four", textNodes.get(2).text());
assertEquals(0, doc.select("br").first().textNodes().size());
}
@Test public void testManipulateTextNodes() {
Document doc = Jsoup.parse("<p>One <span>Two</span> Three <br> Four</p>");
Element p = doc.select("p").first();
List<TextNode> textNodes = p.textNodes();
textNodes.get(1).text(" three-more ");
textNodes.get(2).splitText(3).text("-ur");
assertEquals("One Two three-more Fo-ur", p.text());
assertEquals("One three-more Fo-ur", p.ownText());
assertEquals(4, p.textNodes().size()); // grew because of split
}
@Test public void testGetDataNodes() {
Document doc = Jsoup.parse("<script>One Two</script> <style>Three Four</style> <p>Fix Six</p>");
Element script = doc.select("script").first();
Element style = doc.select("style").first();
Element p = doc.select("p").first();
List<DataNode> scriptData = script.dataNodes();
assertEquals(1, scriptData.size());
assertEquals("One Two", scriptData.get(0).getWholeData());
List<DataNode> styleData = style.dataNodes();
assertEquals(1, styleData.size());
assertEquals("Three Four", styleData.get(0).getWholeData());
List<DataNode> pData = p.dataNodes();
assertEquals(0, pData.size());
}
@Test public void elementIsNotASiblingOfItself() {
Document doc = Jsoup.parse("<div><p>One<p>Two<p>Three</div>");
Element p2 = doc.select("p").get(1);
assertEquals("Two", p2.text());
Elements els = p2.siblingElements();
assertEquals(2, els.size());
assertEquals("<p>One</p>", els.get(0).outerHtml());
assertEquals("<p>Three</p>", els.get(1).outerHtml());
}
@Test public void testChildThrowsIndexOutOfBoundsOnMissing() {
Document doc = Jsoup.parse("<div><p>One</p><p>Two</p></div>");
Element div = doc.select("div").first();
assertEquals(2, div.children().size());
assertEquals("One", div.child(0).text());
try {
div.child(3);
fail("Should throw index out of bounds");
} catch (IndexOutOfBoundsException e) {}
}
@Test
public void moveByAppend() {
// test for https://github.com/jhy/jsoup/issues/239
// can empty an element and append its children to another element
Document doc = Jsoup.parse("<div id=1>Text <p>One</p> Text <p>Two</p></div><div id=2></div>");
Element div1 = doc.select("div").get(0);
Element div2 = doc.select("div").get(1);
assertEquals(4, div1.childNodeSize());
List<Node> children = div1.childNodes();
assertEquals(4, children.size());
div2.insertChildren(0, children);
assertEquals(0, children.size()); // children is backed by div1.childNodes, moved, so should be 0 now
assertEquals(0, div1.childNodeSize());
assertEquals(4, div2.childNodeSize());
assertEquals("<div id=\"1\"></div>\n<div id=\"2\">\n Text \n <p>One</p> Text \n <p>Two</p>\n</div>",
doc.body().html());
}
@Test
public void insertChildrenArgumentValidation() {
Document doc = Jsoup.parse("<div id=1>Text <p>One</p> Text <p>Two</p></div><div id=2></div>");
Element div1 = doc.select("div").get(0);
Element div2 = doc.select("div").get(1);
List<Node> children = div1.childNodes();
try {
div2.insertChildren(6, children);
fail();
} catch (IllegalArgumentException e) {}
try {
div2.insertChildren(-5, children);
fail();
} catch (IllegalArgumentException e) {
}
try {
div2.insertChildren(0, null);
fail();
} catch (IllegalArgumentException e) {
}
}
@Test
public void insertChildrenAtPosition() {
Document doc = Jsoup.parse("<div id=1>Text1 <p>One</p> Text2 <p>Two</p></div><div id=2>Text3 <p>Three</p></div>");
Element div1 = doc.select("div").get(0);
Elements p1s = div1.select("p");
Element div2 = doc.select("div").get(1);
assertEquals(2, div2.childNodeSize());
div2.insertChildren(-1, p1s);
assertEquals(2, div1.childNodeSize()); // moved two out
assertEquals(4, div2.childNodeSize());
assertEquals(3, p1s.get(1).siblingIndex()); // should be last
List<Node> els = new ArrayList<Node>();
Element el1 = new Element(Tag.valueOf("span"), "").text("Span1");
Element el2 = new Element(Tag.valueOf("span"), "").text("Span2");
TextNode tn1 = new TextNode("Text4", "");
els.add(el1);
els.add(el2);
els.add(tn1);
assertNull(el1.parent());
div2.insertChildren(-2, els);
assertEquals(div2, el1.parent());
assertEquals(7, div2.childNodeSize());
assertEquals(3, el1.siblingIndex());
assertEquals(4, el2.siblingIndex());
assertEquals(5, tn1.siblingIndex());
}
@Test
public void insertChildrenAsCopy() {
Document doc = Jsoup.parse("<div id=1>Text <p>One</p> Text <p>Two</p></div><div id=2></div>");
Element div1 = doc.select("div").get(0);
Element div2 = doc.select("div").get(1);
Elements ps = doc.select("p").clone();
ps.first().text("One cloned");
div2.insertChildren(-1, ps);
assertEquals(4, div1.childNodeSize()); // not moved -- cloned
assertEquals(2, div2.childNodeSize());
assertEquals("<div id=\"1\">Text <p>One</p> Text <p>Two</p></div><div id=\"2\"><p>One cloned</p><p>Two</p></div>",
TextUtil.stripNewlines(doc.body().html()));
}
} |
||
public void testParseLocalDate_weekyear_month_week_2010() {
Chronology chrono = GJChronology.getInstanceUTC();
DateTimeFormatter f = DateTimeFormat.forPattern("xxxx-MM-ww").withChronology(chrono);
assertEquals(new LocalDate(2010, 1, 4, chrono), f.parseLocalDate("2010-01-01"));
} | org.joda.time.format.TestDateTimeFormatter::testParseLocalDate_weekyear_month_week_2010 | src/test/java/org/joda/time/format/TestDateTimeFormatter.java | 428 | src/test/java/org/joda/time/format/TestDateTimeFormatter.java | testParseLocalDate_weekyear_month_week_2010 | /*
* Copyright 2001-2011 Stephen Colebourne
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.joda.time.format;
import java.io.CharArrayWriter;
import java.util.Locale;
import java.util.TimeZone;
import junit.framework.TestCase;
import junit.framework.TestSuite;
import org.joda.time.Chronology;
import org.joda.time.DateTime;
import org.joda.time.DateTimeConstants;
import org.joda.time.DateTimeUtils;
import org.joda.time.DateTimeZone;
import org.joda.time.LocalDate;
import org.joda.time.LocalDateTime;
import org.joda.time.LocalTime;
import org.joda.time.MutableDateTime;
import org.joda.time.ReadablePartial;
import org.joda.time.chrono.BuddhistChronology;
import org.joda.time.chrono.GJChronology;
import org.joda.time.chrono.ISOChronology;
/**
* This class is a Junit unit test for DateTime Formating.
*
* @author Stephen Colebourne
*/
public class TestDateTimeFormatter extends TestCase {
private static final DateTimeZone UTC = DateTimeZone.UTC;
private static final DateTimeZone PARIS = DateTimeZone.forID("Europe/Paris");
private static final DateTimeZone LONDON = DateTimeZone.forID("Europe/London");
private static final DateTimeZone TOKYO = DateTimeZone.forID("Asia/Tokyo");
private static final DateTimeZone NEWYORK = DateTimeZone.forID("America/New_York");
private static final Chronology ISO_UTC = ISOChronology.getInstanceUTC();
private static final Chronology ISO_PARIS = ISOChronology.getInstance(PARIS);
private static final Chronology BUDDHIST_PARIS = BuddhistChronology.getInstance(PARIS);
long y2002days = 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 +
365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365;
// 2002-06-09
private long TEST_TIME_NOW =
(y2002days + 31L + 28L + 31L + 30L + 31L + 9L -1L) * DateTimeConstants.MILLIS_PER_DAY;
private DateTimeZone originalDateTimeZone = null;
private TimeZone originalTimeZone = null;
private Locale originalLocale = null;
private DateTimeFormatter f = null;
private DateTimeFormatter g = null;
public static void main(String[] args) {
junit.textui.TestRunner.run(suite());
}
public static TestSuite suite() {
return new TestSuite(TestDateTimeFormatter.class);
}
public TestDateTimeFormatter(String name) {
super(name);
}
protected void setUp() throws Exception {
DateTimeUtils.setCurrentMillisFixed(TEST_TIME_NOW);
originalDateTimeZone = DateTimeZone.getDefault();
originalTimeZone = TimeZone.getDefault();
originalLocale = Locale.getDefault();
DateTimeZone.setDefault(LONDON);
TimeZone.setDefault(TimeZone.getTimeZone("Europe/London"));
Locale.setDefault(Locale.UK);
f = new DateTimeFormatterBuilder()
.appendDayOfWeekShortText()
.appendLiteral(' ')
.append(ISODateTimeFormat.dateTimeNoMillis())
.toFormatter();
g = ISODateTimeFormat.dateTimeNoMillis();
}
protected void tearDown() throws Exception {
DateTimeUtils.setCurrentMillisSystem();
DateTimeZone.setDefault(originalDateTimeZone);
TimeZone.setDefault(originalTimeZone);
Locale.setDefault(originalLocale);
originalDateTimeZone = null;
originalTimeZone = null;
originalLocale = null;
f = null;
g = null;
}
//-----------------------------------------------------------------------
public void testPrint_simple() {
DateTime dt = new DateTime(2004, 6, 9, 10, 20, 30, 40, UTC);
assertEquals("Wed 2004-06-09T10:20:30Z", f.print(dt));
dt = dt.withZone(PARIS);
assertEquals("Wed 2004-06-09T12:20:30+02:00", f.print(dt));
dt = dt.withZone(NEWYORK);
assertEquals("Wed 2004-06-09T06:20:30-04:00", f.print(dt));
dt = dt.withChronology(BUDDHIST_PARIS);
assertEquals("Wed 2547-06-09T12:20:30+02:00", f.print(dt));
}
//-----------------------------------------------------------------------
public void testPrint_locale() {
DateTime dt = new DateTime(2004, 6, 9, 10, 20, 30, 40, UTC);
assertEquals("mer. 2004-06-09T10:20:30Z", f.withLocale(Locale.FRENCH).print(dt));
assertEquals("Wed 2004-06-09T10:20:30Z", f.withLocale(null).print(dt));
}
//-----------------------------------------------------------------------
public void testPrint_zone() {
DateTime dt = new DateTime(2004, 6, 9, 10, 20, 30, 40, UTC);
assertEquals("Wed 2004-06-09T06:20:30-04:00", f.withZone(NEWYORK).print(dt));
assertEquals("Wed 2004-06-09T12:20:30+02:00", f.withZone(PARIS).print(dt));
assertEquals("Wed 2004-06-09T10:20:30Z", f.withZone(null).print(dt));
dt = dt.withZone(NEWYORK);
assertEquals("Wed 2004-06-09T06:20:30-04:00", f.withZone(NEWYORK).print(dt));
assertEquals("Wed 2004-06-09T12:20:30+02:00", f.withZone(PARIS).print(dt));
assertEquals("Wed 2004-06-09T10:20:30Z", f.withZoneUTC().print(dt));
assertEquals("Wed 2004-06-09T06:20:30-04:00", f.withZone(null).print(dt));
}
//-----------------------------------------------------------------------
public void testPrint_chrono() {
DateTime dt = new DateTime(2004, 6, 9, 10, 20, 30, 40, UTC);
assertEquals("Wed 2004-06-09T12:20:30+02:00", f.withChronology(ISO_PARIS).print(dt));
assertEquals("Wed 2547-06-09T12:20:30+02:00", f.withChronology(BUDDHIST_PARIS).print(dt));
assertEquals("Wed 2004-06-09T10:20:30Z", f.withChronology(null).print(dt));
dt = dt.withChronology(BUDDHIST_PARIS);
assertEquals("Wed 2004-06-09T12:20:30+02:00", f.withChronology(ISO_PARIS).print(dt));
assertEquals("Wed 2547-06-09T12:20:30+02:00", f.withChronology(BUDDHIST_PARIS).print(dt));
assertEquals("Wed 2004-06-09T10:20:30Z", f.withChronology(ISO_UTC).print(dt));
assertEquals("Wed 2547-06-09T12:20:30+02:00", f.withChronology(null).print(dt));
}
//-----------------------------------------------------------------------
public void testPrint_bufferMethods() throws Exception {
DateTime dt = new DateTime(2004, 6, 9, 10, 20, 30, 40, UTC);
StringBuffer buf = new StringBuffer();
f.printTo(buf, dt);
assertEquals("Wed 2004-06-09T10:20:30Z", buf.toString());
buf = new StringBuffer();
f.printTo(buf, dt.getMillis());
assertEquals("Wed 2004-06-09T11:20:30+01:00", buf.toString());
buf = new StringBuffer();
ISODateTimeFormat.yearMonthDay().printTo(buf, dt.toYearMonthDay());
assertEquals("2004-06-09", buf.toString());
buf = new StringBuffer();
try {
ISODateTimeFormat.yearMonthDay().printTo(buf, (ReadablePartial) null);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testPrint_writerMethods() throws Exception {
DateTime dt = new DateTime(2004, 6, 9, 10, 20, 30, 40, UTC);
CharArrayWriter out = new CharArrayWriter();
f.printTo(out, dt);
assertEquals("Wed 2004-06-09T10:20:30Z", out.toString());
out = new CharArrayWriter();
f.printTo(out, dt.getMillis());
assertEquals("Wed 2004-06-09T11:20:30+01:00", out.toString());
out = new CharArrayWriter();
ISODateTimeFormat.yearMonthDay().printTo(out, dt.toYearMonthDay());
assertEquals("2004-06-09", out.toString());
out = new CharArrayWriter();
try {
ISODateTimeFormat.yearMonthDay().printTo(out, (ReadablePartial) null);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testPrint_appendableMethods() throws Exception {
DateTime dt = new DateTime(2004, 6, 9, 10, 20, 30, 40, UTC);
StringBuilder buf = new StringBuilder();
f.printTo(buf, dt);
assertEquals("Wed 2004-06-09T10:20:30Z", buf.toString());
buf = new StringBuilder();
f.printTo(buf, dt.getMillis());
assertEquals("Wed 2004-06-09T11:20:30+01:00", buf.toString());
buf = new StringBuilder();
ISODateTimeFormat.yearMonthDay().printTo(buf, dt.toLocalDate());
assertEquals("2004-06-09", buf.toString());
buf = new StringBuilder();
try {
ISODateTimeFormat.yearMonthDay().printTo(buf, (ReadablePartial) null);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testPrint_chrono_and_zone() {
DateTime dt = new DateTime(2004, 6, 9, 10, 20, 30, 40, UTC);
assertEquals("Wed 2004-06-09T10:20:30Z",
f.withChronology(null).withZone(null).print(dt));
assertEquals("Wed 2004-06-09T12:20:30+02:00",
f.withChronology(ISO_PARIS).withZone(null).print(dt));
assertEquals("Wed 2004-06-09T12:20:30+02:00",
f.withChronology(ISO_PARIS).withZone(PARIS).print(dt));
assertEquals("Wed 2004-06-09T06:20:30-04:00",
f.withChronology(ISO_PARIS).withZone(NEWYORK).print(dt));
assertEquals("Wed 2004-06-09T06:20:30-04:00",
f.withChronology(null).withZone(NEWYORK).print(dt));
dt = dt.withChronology(ISO_PARIS);
assertEquals("Wed 2004-06-09T12:20:30+02:00",
f.withChronology(null).withZone(null).print(dt));
assertEquals("Wed 2004-06-09T12:20:30+02:00",
f.withChronology(ISO_PARIS).withZone(null).print(dt));
assertEquals("Wed 2004-06-09T12:20:30+02:00",
f.withChronology(ISO_PARIS).withZone(PARIS).print(dt));
assertEquals("Wed 2004-06-09T06:20:30-04:00",
f.withChronology(ISO_PARIS).withZone(NEWYORK).print(dt));
assertEquals("Wed 2004-06-09T06:20:30-04:00",
f.withChronology(null).withZone(NEWYORK).print(dt));
dt = dt.withChronology(BUDDHIST_PARIS);
assertEquals("Wed 2547-06-09T12:20:30+02:00",
f.withChronology(null).withZone(null).print(dt));
assertEquals("Wed 2004-06-09T12:20:30+02:00",
f.withChronology(ISO_PARIS).withZone(null).print(dt));
assertEquals("Wed 2004-06-09T12:20:30+02:00",
f.withChronology(ISO_PARIS).withZone(PARIS).print(dt));
assertEquals("Wed 2004-06-09T06:20:30-04:00",
f.withChronology(ISO_PARIS).withZone(NEWYORK).print(dt));
assertEquals("Wed 2547-06-09T06:20:30-04:00",
f.withChronology(null).withZone(NEWYORK).print(dt));
}
public void testWithGetLocale() {
DateTimeFormatter f2 = f.withLocale(Locale.FRENCH);
assertEquals(Locale.FRENCH, f2.getLocale());
assertSame(f2, f2.withLocale(Locale.FRENCH));
f2 = f.withLocale(null);
assertEquals(null, f2.getLocale());
assertSame(f2, f2.withLocale(null));
}
public void testWithGetZone() {
DateTimeFormatter f2 = f.withZone(PARIS);
assertEquals(PARIS, f2.getZone());
assertSame(f2, f2.withZone(PARIS));
f2 = f.withZone(null);
assertEquals(null, f2.getZone());
assertSame(f2, f2.withZone(null));
}
public void testWithGetChronology() {
DateTimeFormatter f2 = f.withChronology(BUDDHIST_PARIS);
assertEquals(BUDDHIST_PARIS, f2.getChronology());
assertSame(f2, f2.withChronology(BUDDHIST_PARIS));
f2 = f.withChronology(null);
assertEquals(null, f2.getChronology());
assertSame(f2, f2.withChronology(null));
}
public void testWithGetPivotYear() {
DateTimeFormatter f2 = f.withPivotYear(13);
assertEquals(new Integer(13), f2.getPivotYear());
assertSame(f2, f2.withPivotYear(13));
f2 = f.withPivotYear(new Integer(14));
assertEquals(new Integer(14), f2.getPivotYear());
assertSame(f2, f2.withPivotYear(new Integer(14)));
f2 = f.withPivotYear(null);
assertEquals(null, f2.getPivotYear());
assertSame(f2, f2.withPivotYear(null));
}
public void testWithGetOffsetParsedMethods() {
DateTimeFormatter f2 = f;
assertEquals(false, f2.isOffsetParsed());
assertEquals(null, f2.getZone());
f2 = f.withOffsetParsed();
assertEquals(true, f2.isOffsetParsed());
assertEquals(null, f2.getZone());
f2 = f2.withZone(PARIS);
assertEquals(false, f2.isOffsetParsed());
assertEquals(PARIS, f2.getZone());
f2 = f2.withOffsetParsed();
assertEquals(true, f2.isOffsetParsed());
assertEquals(null, f2.getZone());
f2 = f.withOffsetParsed();
assertNotSame(f, f2);
DateTimeFormatter f3 = f2.withOffsetParsed();
assertSame(f2, f3);
}
public void testPrinterParserMethods() {
DateTimeFormatter f2 = new DateTimeFormatter(f.getPrinter(), f.getParser());
assertEquals(f.getPrinter(), f2.getPrinter());
assertEquals(f.getParser(), f2.getParser());
assertEquals(true, f2.isPrinter());
assertEquals(true, f2.isParser());
assertNotNull(f2.print(0L));
assertNotNull(f2.parseDateTime("Thu 1970-01-01T00:00:00Z"));
f2 = new DateTimeFormatter(f.getPrinter(), null);
assertEquals(f.getPrinter(), f2.getPrinter());
assertEquals(null, f2.getParser());
assertEquals(true, f2.isPrinter());
assertEquals(false, f2.isParser());
assertNotNull(f2.print(0L));
try {
f2.parseDateTime("Thu 1970-01-01T00:00:00Z");
fail();
} catch (UnsupportedOperationException ex) {}
f2 = new DateTimeFormatter(null, f.getParser());
assertEquals(null, f2.getPrinter());
assertEquals(f.getParser(), f2.getParser());
assertEquals(false, f2.isPrinter());
assertEquals(true, f2.isParser());
try {
f2.print(0L);
fail();
} catch (UnsupportedOperationException ex) {}
assertNotNull(f2.parseDateTime("Thu 1970-01-01T00:00:00Z"));
}
//-----------------------------------------------------------------------
public void testParseLocalDate_simple() {
assertEquals(new LocalDate(2004, 6, 9), g.parseLocalDate("2004-06-09T10:20:30Z"));
assertEquals(new LocalDate(2004, 6, 9), g.parseLocalDate("2004-06-09T10:20:30+18:00"));
assertEquals(new LocalDate(2004, 6, 9), g.parseLocalDate("2004-06-09T10:20:30-18:00"));
assertEquals(new LocalDate(2004, 6, 9, BUDDHIST_PARIS),
g.withChronology(BUDDHIST_PARIS).parseLocalDate("2004-06-09T10:20:30Z"));
try {
g.parseDateTime("ABC");
fail();
} catch (IllegalArgumentException ex) {}
}
public void testParseLocalDate_yearOfEra() {
Chronology chrono = GJChronology.getInstanceUTC();
DateTimeFormatter f = DateTimeFormat
.forPattern("YYYY-MM GG")
.withChronology(chrono)
.withLocale(Locale.UK);
LocalDate date = new LocalDate(2005, 10, 1, chrono);
assertEquals(date, f.parseLocalDate("2005-10 AD"));
assertEquals(date, f.parseLocalDate("2005-10 CE"));
date = new LocalDate(-2005, 10, 1, chrono);
assertEquals(date, f.parseLocalDate("2005-10 BC"));
assertEquals(date, f.parseLocalDate("2005-10 BCE"));
}
public void testParseLocalDate_yearOfCentury() {
Chronology chrono = GJChronology.getInstanceUTC();
DateTimeFormatter f = DateTimeFormat
.forPattern("yy M d")
.withChronology(chrono)
.withLocale(Locale.UK)
.withPivotYear(2050);
LocalDate date = new LocalDate(2050, 8, 4, chrono);
assertEquals(date, f.parseLocalDate("50 8 4"));
}
public void testParseLocalDate_monthDay_feb29() {
Chronology chrono = GJChronology.getInstanceUTC();
DateTimeFormatter f = DateTimeFormat
.forPattern("M d")
.withChronology(chrono)
.withLocale(Locale.UK);
assertEquals(new LocalDate(2000, 2, 29, chrono), f.parseLocalDate("2 29"));
}
public void testParseLocalDate_monthDay_withDefaultYear_feb29() {
Chronology chrono = GJChronology.getInstanceUTC();
DateTimeFormatter f = DateTimeFormat
.forPattern("M d")
.withChronology(chrono)
.withLocale(Locale.UK)
.withDefaultYear(2012);
assertEquals(new LocalDate(2012, 2, 29, chrono), f.parseLocalDate("2 29"));
}
public void testParseLocalDate_weekyear_month_week_2010() {
Chronology chrono = GJChronology.getInstanceUTC();
DateTimeFormatter f = DateTimeFormat.forPattern("xxxx-MM-ww").withChronology(chrono);
assertEquals(new LocalDate(2010, 1, 4, chrono), f.parseLocalDate("2010-01-01"));
}
public void testParseLocalDate_weekyear_month_week_2011() {
Chronology chrono = GJChronology.getInstanceUTC();
DateTimeFormatter f = DateTimeFormat.forPattern("xxxx-MM-ww").withChronology(chrono);
assertEquals(new LocalDate(2011, 1, 3, chrono), f.parseLocalDate("2011-01-01"));
}
public void testParseLocalDate_weekyear_month_week_2012() {
Chronology chrono = GJChronology.getInstanceUTC();
DateTimeFormatter f = DateTimeFormat.forPattern("xxxx-MM-ww").withChronology(chrono);
assertEquals(new LocalDate(2012, 1, 2, chrono), f.parseLocalDate("2012-01-01"));
}
// This test fails, but since more related tests pass with the extra loop in DateTimeParserBucket
// I'm going to leave the change in and ignore this test
// public void testParseLocalDate_weekyear_month_week_2013() {
// Chronology chrono = GJChronology.getInstanceUTC();
// DateTimeFormatter f = DateTimeFormat.forPattern("xxxx-MM-ww").withChronology(chrono);
// assertEquals(new LocalDate(2012, 12, 31, chrono), f.parseLocalDate("2013-01-01"));
// }
public void testParseLocalDate_year_month_week_2010() {
Chronology chrono = GJChronology.getInstanceUTC();
DateTimeFormatter f = DateTimeFormat.forPattern("yyyy-MM-ww").withChronology(chrono);
assertEquals(new LocalDate(2010, 1, 4, chrono), f.parseLocalDate("2010-01-01"));
}
public void testParseLocalDate_year_month_week_2011() {
Chronology chrono = GJChronology.getInstanceUTC();
DateTimeFormatter f = DateTimeFormat.forPattern("yyyy-MM-ww").withChronology(chrono);
assertEquals(new LocalDate(2011, 1, 3, chrono), f.parseLocalDate("2011-01-01"));
}
public void testParseLocalDate_year_month_week_2012() {
Chronology chrono = GJChronology.getInstanceUTC();
DateTimeFormatter f = DateTimeFormat.forPattern("yyyy-MM-ww").withChronology(chrono);
assertEquals(new LocalDate(2012, 1, 2, chrono), f.parseLocalDate("2012-01-01"));
}
public void testParseLocalDate_year_month_week_2013() {
Chronology chrono = GJChronology.getInstanceUTC();
DateTimeFormatter f = DateTimeFormat.forPattern("yyyy-MM-ww").withChronology(chrono);
assertEquals(new LocalDate(2012, 12, 31, chrono), f.parseLocalDate("2013-01-01")); // 2013-01-01 would be better, but this is OK
}
public void testParseLocalDate_year_month_week_2014() {
Chronology chrono = GJChronology.getInstanceUTC();
DateTimeFormatter f = DateTimeFormat.forPattern("yyyy-MM-ww").withChronology(chrono);
assertEquals(new LocalDate(2013, 12, 30, chrono), f.parseLocalDate("2014-01-01")); // 2014-01-01 would be better, but this is OK
}
public void testParseLocalDate_year_month_week_2015() {
Chronology chrono = GJChronology.getInstanceUTC();
DateTimeFormatter f = DateTimeFormat.forPattern("yyyy-MM-ww").withChronology(chrono);
assertEquals(new LocalDate(2014, 12, 29, chrono), f.parseLocalDate("2015-01-01")); // 2015-01-01 would be better, but this is OK
}
public void testParseLocalDate_year_month_week_2016() {
Chronology chrono = GJChronology.getInstanceUTC();
DateTimeFormatter f = DateTimeFormat.forPattern("yyyy-MM-ww").withChronology(chrono);
assertEquals(new LocalDate(2016, 1, 4, chrono), f.parseLocalDate("2016-01-01"));
}
//-----------------------------------------------------------------------
public void testParseLocalTime_simple() {
assertEquals(new LocalTime(10, 20, 30), g.parseLocalTime("2004-06-09T10:20:30Z"));
assertEquals(new LocalTime(10, 20, 30), g.parseLocalTime("2004-06-09T10:20:30+18:00"));
assertEquals(new LocalTime(10, 20, 30), g.parseLocalTime("2004-06-09T10:20:30-18:00"));
assertEquals(new LocalTime(10, 20, 30, 0, BUDDHIST_PARIS),
g.withChronology(BUDDHIST_PARIS).parseLocalTime("2004-06-09T10:20:30Z"));
try {
g.parseDateTime("ABC");
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testParseLocalDateTime_simple() {
assertEquals(new LocalDateTime(2004, 6, 9, 10, 20, 30), g.parseLocalDateTime("2004-06-09T10:20:30Z"));
assertEquals(new LocalDateTime(2004, 6, 9, 10, 20, 30), g.parseLocalDateTime("2004-06-09T10:20:30+18:00"));
assertEquals(new LocalDateTime(2004, 6, 9, 10, 20, 30), g.parseLocalDateTime("2004-06-09T10:20:30-18:00"));
assertEquals(new LocalDateTime(2004, 6, 9, 10, 20, 30, 0, BUDDHIST_PARIS),
g.withChronology(BUDDHIST_PARIS).parseLocalDateTime("2004-06-09T10:20:30Z"));
try {
g.parseDateTime("ABC");
fail();
} catch (IllegalArgumentException ex) {}
}
public void testParseLocalDateTime_monthDay_feb29() {
Chronology chrono = GJChronology.getInstanceUTC();
DateTimeFormatter f = DateTimeFormat
.forPattern("M d H m")
.withChronology(chrono)
.withLocale(Locale.UK);
assertEquals(new LocalDateTime(2000, 2, 29, 13, 40, 0, 0, chrono), f.parseLocalDateTime("2 29 13 40"));
}
public void testParseLocalDateTime_monthDay_withDefaultYear_feb29() {
Chronology chrono = GJChronology.getInstanceUTC();
DateTimeFormatter f = DateTimeFormat
.forPattern("M d H m")
.withChronology(chrono)
.withLocale(Locale.UK)
.withDefaultYear(2012);
assertEquals(new LocalDateTime(2012, 2, 29, 13, 40, 0, 0, chrono), f.parseLocalDateTime("2 29 13 40"));
}
//-----------------------------------------------------------------------
public void testParseDateTime_simple() {
DateTime expect = null;
expect = new DateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
assertEquals(expect, g.parseDateTime("2004-06-09T10:20:30Z"));
try {
g.parseDateTime("ABC");
fail();
} catch (IllegalArgumentException ex) {}
}
public void testParseDateTime_zone() {
DateTime expect = null;
expect = new DateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
assertEquals(expect, g.withZone(LONDON).parseDateTime("2004-06-09T10:20:30Z"));
expect = new DateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
assertEquals(expect, g.withZone(null).parseDateTime("2004-06-09T10:20:30Z"));
expect = new DateTime(2004, 6, 9, 12, 20, 30, 0, PARIS);
assertEquals(expect, g.withZone(PARIS).parseDateTime("2004-06-09T10:20:30Z"));
}
public void testParseDateTime_zone2() {
DateTime expect = null;
expect = new DateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
assertEquals(expect, g.withZone(LONDON).parseDateTime("2004-06-09T06:20:30-04:00"));
expect = new DateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
assertEquals(expect, g.withZone(null).parseDateTime("2004-06-09T06:20:30-04:00"));
expect = new DateTime(2004, 6, 9, 12, 20, 30, 0, PARIS);
assertEquals(expect, g.withZone(PARIS).parseDateTime("2004-06-09T06:20:30-04:00"));
}
public void testParseDateTime_zone3() {
DateTimeFormatter h = new DateTimeFormatterBuilder()
.append(ISODateTimeFormat.date())
.appendLiteral('T')
.append(ISODateTimeFormat.timeElementParser())
.toFormatter();
DateTime expect = null;
expect = new DateTime(2004, 6, 9, 10, 20, 30, 0, LONDON);
assertEquals(expect, h.withZone(LONDON).parseDateTime("2004-06-09T10:20:30"));
expect = new DateTime(2004, 6, 9, 10, 20, 30, 0, LONDON);
assertEquals(expect, h.withZone(null).parseDateTime("2004-06-09T10:20:30"));
expect = new DateTime(2004, 6, 9, 10, 20, 30, 0, PARIS);
assertEquals(expect, h.withZone(PARIS).parseDateTime("2004-06-09T10:20:30"));
}
public void testParseDateTime_simple_precedence() {
DateTime expect = null;
// use correct day of week
expect = new DateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
assertEquals(expect, f.parseDateTime("Wed 2004-06-09T10:20:30Z"));
// use wrong day of week
expect = new DateTime(2004, 6, 7, 11, 20, 30, 0, LONDON);
// DayOfWeek takes precedence, because week < month in length
assertEquals(expect, f.parseDateTime("Mon 2004-06-09T10:20:30Z"));
}
public void testParseDateTime_offsetParsed() {
DateTime expect = null;
expect = new DateTime(2004, 6, 9, 10, 20, 30, 0, UTC);
assertEquals(expect, g.withOffsetParsed().parseDateTime("2004-06-09T10:20:30Z"));
expect = new DateTime(2004, 6, 9, 6, 20, 30, 0, DateTimeZone.forOffsetHours(-4));
assertEquals(expect, g.withOffsetParsed().parseDateTime("2004-06-09T06:20:30-04:00"));
expect = new DateTime(2004, 6, 9, 10, 20, 30, 0, UTC);
assertEquals(expect, g.withZone(PARIS).withOffsetParsed().parseDateTime("2004-06-09T10:20:30Z"));
expect = new DateTime(2004, 6, 9, 12, 20, 30, 0, PARIS);
assertEquals(expect, g.withOffsetParsed().withZone(PARIS).parseDateTime("2004-06-09T10:20:30Z"));
}
public void testParseDateTime_chrono() {
DateTime expect = null;
expect = new DateTime(2004, 6, 9, 12, 20, 30, 0, PARIS);
assertEquals(expect, g.withChronology(ISO_PARIS).parseDateTime("2004-06-09T10:20:30Z"));
expect = new DateTime(2004, 6, 9, 11, 20, 30, 0,LONDON);
assertEquals(expect, g.withChronology(null).parseDateTime("2004-06-09T10:20:30Z"));
expect = new DateTime(2547, 6, 9, 12, 20, 30, 0, BUDDHIST_PARIS);
assertEquals(expect, g.withChronology(BUDDHIST_PARIS).parseDateTime("2547-06-09T10:20:30Z"));
expect = new DateTime(2004, 6, 9, 10, 29, 51, 0, BUDDHIST_PARIS); // zone is +00:09:21 in 1451
assertEquals(expect, g.withChronology(BUDDHIST_PARIS).parseDateTime("2004-06-09T10:20:30Z"));
}
//-----------------------------------------------------------------------
public void testParseMutableDateTime_simple() {
MutableDateTime expect = null;
expect = new MutableDateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
assertEquals(expect, g.parseMutableDateTime("2004-06-09T10:20:30Z"));
try {
g.parseMutableDateTime("ABC");
fail();
} catch (IllegalArgumentException ex) {}
}
public void testParseMutableDateTime_zone() {
MutableDateTime expect = null;
expect = new MutableDateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
assertEquals(expect, g.withZone(LONDON).parseMutableDateTime("2004-06-09T10:20:30Z"));
expect = new MutableDateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
assertEquals(expect, g.withZone(null).parseMutableDateTime("2004-06-09T10:20:30Z"));
expect = new MutableDateTime(2004, 6, 9, 12, 20, 30, 0, PARIS);
assertEquals(expect, g.withZone(PARIS).parseMutableDateTime("2004-06-09T10:20:30Z"));
}
public void testParseMutableDateTime_zone2() {
MutableDateTime expect = null;
expect = new MutableDateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
assertEquals(expect, g.withZone(LONDON).parseMutableDateTime("2004-06-09T06:20:30-04:00"));
expect = new MutableDateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
assertEquals(expect, g.withZone(null).parseMutableDateTime("2004-06-09T06:20:30-04:00"));
expect = new MutableDateTime(2004, 6, 9, 12, 20, 30, 0, PARIS);
assertEquals(expect, g.withZone(PARIS).parseMutableDateTime("2004-06-09T06:20:30-04:00"));
}
public void testParseMutableDateTime_zone3() {
DateTimeFormatter h = new DateTimeFormatterBuilder()
.append(ISODateTimeFormat.date())
.appendLiteral('T')
.append(ISODateTimeFormat.timeElementParser())
.toFormatter();
MutableDateTime expect = null;
expect = new MutableDateTime(2004, 6, 9, 10, 20, 30, 0, LONDON);
assertEquals(expect, h.withZone(LONDON).parseMutableDateTime("2004-06-09T10:20:30"));
expect = new MutableDateTime(2004, 6, 9, 10, 20, 30, 0, LONDON);
assertEquals(expect, h.withZone(null).parseMutableDateTime("2004-06-09T10:20:30"));
expect = new MutableDateTime(2004, 6, 9, 10, 20, 30, 0, PARIS);
assertEquals(expect, h.withZone(PARIS).parseMutableDateTime("2004-06-09T10:20:30"));
}
public void testParseMutableDateTime_simple_precedence() {
MutableDateTime expect = null;
// use correct day of week
expect = new MutableDateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
assertEquals(expect, f.parseDateTime("Wed 2004-06-09T10:20:30Z"));
// use wrong day of week
expect = new MutableDateTime(2004, 6, 7, 11, 20, 30, 0, LONDON);
// DayOfWeek takes precedence, because week < month in length
assertEquals(expect, f.parseDateTime("Mon 2004-06-09T10:20:30Z"));
}
public void testParseMutableDateTime_offsetParsed() {
MutableDateTime expect = null;
expect = new MutableDateTime(2004, 6, 9, 10, 20, 30, 0, UTC);
assertEquals(expect, g.withOffsetParsed().parseMutableDateTime("2004-06-09T10:20:30Z"));
expect = new MutableDateTime(2004, 6, 9, 6, 20, 30, 0, DateTimeZone.forOffsetHours(-4));
assertEquals(expect, g.withOffsetParsed().parseMutableDateTime("2004-06-09T06:20:30-04:00"));
expect = new MutableDateTime(2004, 6, 9, 10, 20, 30, 0, UTC);
assertEquals(expect, g.withZone(PARIS).withOffsetParsed().parseMutableDateTime("2004-06-09T10:20:30Z"));
expect = new MutableDateTime(2004, 6, 9, 12, 20, 30, 0, PARIS);
assertEquals(expect, g.withOffsetParsed().withZone(PARIS).parseMutableDateTime("2004-06-09T10:20:30Z"));
}
public void testParseMutableDateTime_chrono() {
MutableDateTime expect = null;
expect = new MutableDateTime(2004, 6, 9, 12, 20, 30, 0, PARIS);
assertEquals(expect, g.withChronology(ISO_PARIS).parseMutableDateTime("2004-06-09T10:20:30Z"));
expect = new MutableDateTime(2004, 6, 9, 11, 20, 30, 0,LONDON);
assertEquals(expect, g.withChronology(null).parseMutableDateTime("2004-06-09T10:20:30Z"));
expect = new MutableDateTime(2547, 6, 9, 12, 20, 30, 0, BUDDHIST_PARIS);
assertEquals(expect, g.withChronology(BUDDHIST_PARIS).parseMutableDateTime("2547-06-09T10:20:30Z"));
expect = new MutableDateTime(2004, 6, 9, 10, 29, 51, 0, BUDDHIST_PARIS); // zone is +00:09:21 in 1451
assertEquals(expect, g.withChronology(BUDDHIST_PARIS).parseMutableDateTime("2004-06-09T10:20:30Z"));
}
//-----------------------------------------------------------------------
public void testParseInto_simple() {
MutableDateTime expect = null;
expect = new MutableDateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
MutableDateTime result = new MutableDateTime(0L);
assertEquals(20, g.parseInto(result, "2004-06-09T10:20:30Z", 0));
assertEquals(expect, result);
try {
g.parseInto(null, "2004-06-09T10:20:30Z", 0);
fail();
} catch (IllegalArgumentException ex) {}
assertEquals(~0, g.parseInto(result, "ABC", 0));
assertEquals(~10, g.parseInto(result, "2004-06-09", 0));
assertEquals(~13, g.parseInto(result, "XX2004-06-09T", 2));
}
public void testParseInto_zone() {
MutableDateTime expect = null;
MutableDateTime result = null;
expect = new MutableDateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
result = new MutableDateTime(0L);
assertEquals(20, g.withZone(LONDON).parseInto(result, "2004-06-09T10:20:30Z", 0));
assertEquals(expect, result);
expect = new MutableDateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
result = new MutableDateTime(0L);
assertEquals(20, g.withZone(null).parseInto(result, "2004-06-09T10:20:30Z", 0));
assertEquals(expect, result);
expect = new MutableDateTime(2004, 6, 9, 12, 20, 30, 0, PARIS);
result = new MutableDateTime(0L);
assertEquals(20, g.withZone(PARIS).parseInto(result, "2004-06-09T10:20:30Z", 0));
assertEquals(expect, result);
}
public void testParseInto_zone2() {
MutableDateTime expect = null;
MutableDateTime result = null;
expect = new MutableDateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
result = new MutableDateTime(0L);
assertEquals(25, g.withZone(LONDON).parseInto(result, "2004-06-09T06:20:30-04:00", 0));
assertEquals(expect, result);
expect = new MutableDateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
assertEquals(25, g.withZone(null).parseInto(result, "2004-06-09T06:20:30-04:00", 0));
assertEquals(expect, result);
expect = new MutableDateTime(2004, 6, 9, 12, 20, 30, 0, PARIS);
assertEquals(25, g.withZone(PARIS).parseInto(result, "2004-06-09T06:20:30-04:00", 0));
assertEquals(expect, result);
}
public void testParseInto_zone3() {
DateTimeFormatter h = new DateTimeFormatterBuilder()
.append(ISODateTimeFormat.date())
.appendLiteral('T')
.append(ISODateTimeFormat.timeElementParser())
.toFormatter();
MutableDateTime expect = null;
MutableDateTime result = null;
expect = new MutableDateTime(2004, 6, 9, 10, 20, 30, 0, LONDON);
result = new MutableDateTime(0L);
assertEquals(19, h.withZone(LONDON).parseInto(result, "2004-06-09T10:20:30", 0));
assertEquals(expect, result);
expect = new MutableDateTime(2004, 6, 9, 10, 20, 30, 0, LONDON);
result = new MutableDateTime(0L);
assertEquals(19, h.withZone(null).parseInto(result, "2004-06-09T10:20:30", 0));
assertEquals(expect, result);
expect = new MutableDateTime(2004, 6, 9, 10, 20, 30, 0, PARIS);
result = new MutableDateTime(0L);
assertEquals(19, h.withZone(PARIS).parseInto(result, "2004-06-09T10:20:30", 0));
assertEquals(expect, result);
}
public void testParseInto_simple_precedence() {
MutableDateTime expect = null;
MutableDateTime result = null;
expect = new MutableDateTime(2004, 6, 7, 11, 20, 30, 0, LONDON);
result = new MutableDateTime(0L);
// DayOfWeek takes precedence, because week < month in length
assertEquals(24, f.parseInto(result, "Mon 2004-06-09T10:20:30Z", 0));
assertEquals(expect, result);
}
public void testParseInto_offsetParsed() {
MutableDateTime expect = null;
MutableDateTime result = null;
expect = new MutableDateTime(2004, 6, 9, 10, 20, 30, 0, UTC);
result = new MutableDateTime(0L);
assertEquals(20, g.withOffsetParsed().parseInto(result, "2004-06-09T10:20:30Z", 0));
assertEquals(expect, result);
expect = new MutableDateTime(2004, 6, 9, 6, 20, 30, 0, DateTimeZone.forOffsetHours(-4));
result = new MutableDateTime(0L);
assertEquals(25, g.withOffsetParsed().parseInto(result, "2004-06-09T06:20:30-04:00", 0));
assertEquals(expect, result);
expect = new MutableDateTime(2004, 6, 9, 10, 20, 30, 0, UTC);
result = new MutableDateTime(0L);
assertEquals(20, g.withZone(PARIS).withOffsetParsed().parseInto(result, "2004-06-09T10:20:30Z", 0));
assertEquals(expect, result);
expect = new MutableDateTime(2004, 6, 9, 12, 20, 30, 0, PARIS);
result = new MutableDateTime(0L);
assertEquals(20, g.withOffsetParsed().withZone(PARIS).parseInto(result, "2004-06-09T10:20:30Z", 0));
assertEquals(expect, result);
}
public void testParseInto_chrono() {
MutableDateTime expect = null;
MutableDateTime result = null;
expect = new MutableDateTime(2004, 6, 9, 12, 20, 30, 0, PARIS);
result = new MutableDateTime(0L);
assertEquals(20, g.withChronology(ISO_PARIS).parseInto(result, "2004-06-09T10:20:30Z", 0));
assertEquals(expect, result);
expect = new MutableDateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
result = new MutableDateTime(0L);
assertEquals(20, g.withChronology(null).parseInto(result, "2004-06-09T10:20:30Z", 0));
assertEquals(expect, result);
expect = new MutableDateTime(2547, 6, 9, 12, 20, 30, 0, BUDDHIST_PARIS);
result = new MutableDateTime(0L);
assertEquals(20, g.withChronology(BUDDHIST_PARIS).parseInto(result, "2547-06-09T10:20:30Z", 0));
assertEquals(expect, result);
expect = new MutableDateTime(2004, 6, 9, 10, 29, 51, 0, BUDDHIST_PARIS);
result = new MutableDateTime(0L);
assertEquals(20, g.withChronology(BUDDHIST_PARIS).parseInto(result, "2004-06-09T10:20:30Z", 0));
assertEquals(expect, result);
}
public void testParseMillis_fractionOfSecondLong() {
DateTimeFormatter f = new DateTimeFormatterBuilder()
.appendSecondOfDay(2).appendLiteral('.').appendFractionOfSecond(1, 9)
.toFormatter().withZoneUTC();
assertEquals(10512, f.parseMillis("10.5123456"));
assertEquals(10512, f.parseMillis("10.512999"));
}
//-----------------------------------------------------------------------
// Ensure time zone name switches properly at the zone DST transition.
public void testZoneNameNearTransition() {}
// Defects4J: flaky method
// public void testZoneNameNearTransition() {
// DateTime inDST_1 = new DateTime(2005, 10, 30, 1, 0, 0, 0, NEWYORK);
// DateTime inDST_2 = new DateTime(2005, 10, 30, 1, 59, 59, 999, NEWYORK);
// DateTime onDST = new DateTime(2005, 10, 30, 2, 0, 0, 0, NEWYORK);
// DateTime outDST = new DateTime(2005, 10, 30, 2, 0, 0, 1, NEWYORK);
// DateTime outDST_2 = new DateTime(2005, 10, 30, 2, 0, 1, 0, NEWYORK);
//
// DateTimeFormatter fmt = DateTimeFormat.forPattern("yyy-MM-dd HH:mm:ss.S zzzz");
// assertEquals("2005-10-30 01:00:00.0 Eastern Daylight Time", fmt.print(inDST_1));
// assertEquals("2005-10-30 01:59:59.9 Eastern Daylight Time", fmt.print(inDST_2));
// assertEquals("2005-10-30 02:00:00.0 Eastern Standard Time", fmt.print(onDST));
// assertEquals("2005-10-30 02:00:00.0 Eastern Standard Time", fmt.print(outDST));
// assertEquals("2005-10-30 02:00:01.0 Eastern Standard Time", fmt.print(outDST_2));
// }
// Ensure time zone name switches properly at the zone DST transition.
public void testZoneShortNameNearTransition() {}
// Defects4J: flaky method
// public void testZoneShortNameNearTransition() {
// DateTime inDST_1 = new DateTime(2005, 10, 30, 1, 0, 0, 0, NEWYORK);
// DateTime inDST_2 = new DateTime(2005, 10, 30, 1, 59, 59, 999, NEWYORK);
// DateTime onDST = new DateTime(2005, 10, 30, 2, 0, 0, 0, NEWYORK);
// DateTime outDST = new DateTime(2005, 10, 30, 2, 0, 0, 1, NEWYORK);
// DateTime outDST_2 = new DateTime(2005, 10, 30, 2, 0, 1, 0, NEWYORK);
//
// DateTimeFormatter fmt = DateTimeFormat.forPattern("yyy-MM-dd HH:mm:ss.S z");
// assertEquals("2005-10-30 01:00:00.0 EDT", fmt.print(inDST_1));
// assertEquals("2005-10-30 01:59:59.9 EDT", fmt.print(inDST_2));
// assertEquals("2005-10-30 02:00:00.0 EST", fmt.print(onDST));
// assertEquals("2005-10-30 02:00:00.0 EST", fmt.print(outDST));
// assertEquals("2005-10-30 02:00:01.0 EST", fmt.print(outDST_2));
// }
} | // You are a professional Java test case writer, please create a test case named `testParseLocalDate_weekyear_month_week_2010` for the issue `Time-107`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Time-107
//
// ## Issue-Title:
// #107 Incorrect date parsed when week and month used together
//
//
//
//
//
// ## Issue-Description:
// I have following code snippet :
//
//
//
// ```
// DateTimeFormatter dtf = DateTimeFormat.forPattern("xxxxMM'w'ww");
// DateTime dt = dtf.parseDateTime("201101w01");
// System.out.println(dt);
//
// ```
//
// It should print 2011-01-03 but it is printing 2010-01-04.
//
// Please let me know if I am doing something wrong here.
//
//
//
//
public void testParseLocalDate_weekyear_month_week_2010() {
| 428 | 24 | 424 | src/test/java/org/joda/time/format/TestDateTimeFormatter.java | src/test/java | ```markdown
## Issue-ID: Time-107
## Issue-Title:
#107 Incorrect date parsed when week and month used together
## Issue-Description:
I have following code snippet :
```
DateTimeFormatter dtf = DateTimeFormat.forPattern("xxxxMM'w'ww");
DateTime dt = dtf.parseDateTime("201101w01");
System.out.println(dt);
```
It should print 2011-01-03 but it is printing 2010-01-04.
Please let me know if I am doing something wrong here.
```
You are a professional Java test case writer, please create a test case named `testParseLocalDate_weekyear_month_week_2010` for the issue `Time-107`, utilizing the provided issue report information and the following function signature.
```java
public void testParseLocalDate_weekyear_month_week_2010() {
```
| 424 | [
"org.joda.time.format.DateTimeParserBucket"
] | 7d8784779bd09c304f2cf70f9bb0e1d2494e77e153c91b5a26ae4964a4a07d24 | public void testParseLocalDate_weekyear_month_week_2010() | // You are a professional Java test case writer, please create a test case named `testParseLocalDate_weekyear_month_week_2010` for the issue `Time-107`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Time-107
//
// ## Issue-Title:
// #107 Incorrect date parsed when week and month used together
//
//
//
//
//
// ## Issue-Description:
// I have following code snippet :
//
//
//
// ```
// DateTimeFormatter dtf = DateTimeFormat.forPattern("xxxxMM'w'ww");
// DateTime dt = dtf.parseDateTime("201101w01");
// System.out.println(dt);
//
// ```
//
// It should print 2011-01-03 but it is printing 2010-01-04.
//
// Please let me know if I am doing something wrong here.
//
//
//
//
| Time | /*
* Copyright 2001-2011 Stephen Colebourne
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.joda.time.format;
import java.io.CharArrayWriter;
import java.util.Locale;
import java.util.TimeZone;
import junit.framework.TestCase;
import junit.framework.TestSuite;
import org.joda.time.Chronology;
import org.joda.time.DateTime;
import org.joda.time.DateTimeConstants;
import org.joda.time.DateTimeUtils;
import org.joda.time.DateTimeZone;
import org.joda.time.LocalDate;
import org.joda.time.LocalDateTime;
import org.joda.time.LocalTime;
import org.joda.time.MutableDateTime;
import org.joda.time.ReadablePartial;
import org.joda.time.chrono.BuddhistChronology;
import org.joda.time.chrono.GJChronology;
import org.joda.time.chrono.ISOChronology;
/**
* This class is a Junit unit test for DateTime Formating.
*
* @author Stephen Colebourne
*/
public class TestDateTimeFormatter extends TestCase {
private static final DateTimeZone UTC = DateTimeZone.UTC;
private static final DateTimeZone PARIS = DateTimeZone.forID("Europe/Paris");
private static final DateTimeZone LONDON = DateTimeZone.forID("Europe/London");
private static final DateTimeZone TOKYO = DateTimeZone.forID("Asia/Tokyo");
private static final DateTimeZone NEWYORK = DateTimeZone.forID("America/New_York");
private static final Chronology ISO_UTC = ISOChronology.getInstanceUTC();
private static final Chronology ISO_PARIS = ISOChronology.getInstance(PARIS);
private static final Chronology BUDDHIST_PARIS = BuddhistChronology.getInstance(PARIS);
long y2002days = 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 +
365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365;
// 2002-06-09
private long TEST_TIME_NOW =
(y2002days + 31L + 28L + 31L + 30L + 31L + 9L -1L) * DateTimeConstants.MILLIS_PER_DAY;
private DateTimeZone originalDateTimeZone = null;
private TimeZone originalTimeZone = null;
private Locale originalLocale = null;
private DateTimeFormatter f = null;
private DateTimeFormatter g = null;
public static void main(String[] args) {
junit.textui.TestRunner.run(suite());
}
public static TestSuite suite() {
return new TestSuite(TestDateTimeFormatter.class);
}
public TestDateTimeFormatter(String name) {
super(name);
}
protected void setUp() throws Exception {
DateTimeUtils.setCurrentMillisFixed(TEST_TIME_NOW);
originalDateTimeZone = DateTimeZone.getDefault();
originalTimeZone = TimeZone.getDefault();
originalLocale = Locale.getDefault();
DateTimeZone.setDefault(LONDON);
TimeZone.setDefault(TimeZone.getTimeZone("Europe/London"));
Locale.setDefault(Locale.UK);
f = new DateTimeFormatterBuilder()
.appendDayOfWeekShortText()
.appendLiteral(' ')
.append(ISODateTimeFormat.dateTimeNoMillis())
.toFormatter();
g = ISODateTimeFormat.dateTimeNoMillis();
}
protected void tearDown() throws Exception {
DateTimeUtils.setCurrentMillisSystem();
DateTimeZone.setDefault(originalDateTimeZone);
TimeZone.setDefault(originalTimeZone);
Locale.setDefault(originalLocale);
originalDateTimeZone = null;
originalTimeZone = null;
originalLocale = null;
f = null;
g = null;
}
//-----------------------------------------------------------------------
public void testPrint_simple() {
DateTime dt = new DateTime(2004, 6, 9, 10, 20, 30, 40, UTC);
assertEquals("Wed 2004-06-09T10:20:30Z", f.print(dt));
dt = dt.withZone(PARIS);
assertEquals("Wed 2004-06-09T12:20:30+02:00", f.print(dt));
dt = dt.withZone(NEWYORK);
assertEquals("Wed 2004-06-09T06:20:30-04:00", f.print(dt));
dt = dt.withChronology(BUDDHIST_PARIS);
assertEquals("Wed 2547-06-09T12:20:30+02:00", f.print(dt));
}
//-----------------------------------------------------------------------
public void testPrint_locale() {
DateTime dt = new DateTime(2004, 6, 9, 10, 20, 30, 40, UTC);
assertEquals("mer. 2004-06-09T10:20:30Z", f.withLocale(Locale.FRENCH).print(dt));
assertEquals("Wed 2004-06-09T10:20:30Z", f.withLocale(null).print(dt));
}
//-----------------------------------------------------------------------
public void testPrint_zone() {
DateTime dt = new DateTime(2004, 6, 9, 10, 20, 30, 40, UTC);
assertEquals("Wed 2004-06-09T06:20:30-04:00", f.withZone(NEWYORK).print(dt));
assertEquals("Wed 2004-06-09T12:20:30+02:00", f.withZone(PARIS).print(dt));
assertEquals("Wed 2004-06-09T10:20:30Z", f.withZone(null).print(dt));
dt = dt.withZone(NEWYORK);
assertEquals("Wed 2004-06-09T06:20:30-04:00", f.withZone(NEWYORK).print(dt));
assertEquals("Wed 2004-06-09T12:20:30+02:00", f.withZone(PARIS).print(dt));
assertEquals("Wed 2004-06-09T10:20:30Z", f.withZoneUTC().print(dt));
assertEquals("Wed 2004-06-09T06:20:30-04:00", f.withZone(null).print(dt));
}
//-----------------------------------------------------------------------
public void testPrint_chrono() {
DateTime dt = new DateTime(2004, 6, 9, 10, 20, 30, 40, UTC);
assertEquals("Wed 2004-06-09T12:20:30+02:00", f.withChronology(ISO_PARIS).print(dt));
assertEquals("Wed 2547-06-09T12:20:30+02:00", f.withChronology(BUDDHIST_PARIS).print(dt));
assertEquals("Wed 2004-06-09T10:20:30Z", f.withChronology(null).print(dt));
dt = dt.withChronology(BUDDHIST_PARIS);
assertEquals("Wed 2004-06-09T12:20:30+02:00", f.withChronology(ISO_PARIS).print(dt));
assertEquals("Wed 2547-06-09T12:20:30+02:00", f.withChronology(BUDDHIST_PARIS).print(dt));
assertEquals("Wed 2004-06-09T10:20:30Z", f.withChronology(ISO_UTC).print(dt));
assertEquals("Wed 2547-06-09T12:20:30+02:00", f.withChronology(null).print(dt));
}
//-----------------------------------------------------------------------
public void testPrint_bufferMethods() throws Exception {
DateTime dt = new DateTime(2004, 6, 9, 10, 20, 30, 40, UTC);
StringBuffer buf = new StringBuffer();
f.printTo(buf, dt);
assertEquals("Wed 2004-06-09T10:20:30Z", buf.toString());
buf = new StringBuffer();
f.printTo(buf, dt.getMillis());
assertEquals("Wed 2004-06-09T11:20:30+01:00", buf.toString());
buf = new StringBuffer();
ISODateTimeFormat.yearMonthDay().printTo(buf, dt.toYearMonthDay());
assertEquals("2004-06-09", buf.toString());
buf = new StringBuffer();
try {
ISODateTimeFormat.yearMonthDay().printTo(buf, (ReadablePartial) null);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testPrint_writerMethods() throws Exception {
DateTime dt = new DateTime(2004, 6, 9, 10, 20, 30, 40, UTC);
CharArrayWriter out = new CharArrayWriter();
f.printTo(out, dt);
assertEquals("Wed 2004-06-09T10:20:30Z", out.toString());
out = new CharArrayWriter();
f.printTo(out, dt.getMillis());
assertEquals("Wed 2004-06-09T11:20:30+01:00", out.toString());
out = new CharArrayWriter();
ISODateTimeFormat.yearMonthDay().printTo(out, dt.toYearMonthDay());
assertEquals("2004-06-09", out.toString());
out = new CharArrayWriter();
try {
ISODateTimeFormat.yearMonthDay().printTo(out, (ReadablePartial) null);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testPrint_appendableMethods() throws Exception {
DateTime dt = new DateTime(2004, 6, 9, 10, 20, 30, 40, UTC);
StringBuilder buf = new StringBuilder();
f.printTo(buf, dt);
assertEquals("Wed 2004-06-09T10:20:30Z", buf.toString());
buf = new StringBuilder();
f.printTo(buf, dt.getMillis());
assertEquals("Wed 2004-06-09T11:20:30+01:00", buf.toString());
buf = new StringBuilder();
ISODateTimeFormat.yearMonthDay().printTo(buf, dt.toLocalDate());
assertEquals("2004-06-09", buf.toString());
buf = new StringBuilder();
try {
ISODateTimeFormat.yearMonthDay().printTo(buf, (ReadablePartial) null);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testPrint_chrono_and_zone() {
DateTime dt = new DateTime(2004, 6, 9, 10, 20, 30, 40, UTC);
assertEquals("Wed 2004-06-09T10:20:30Z",
f.withChronology(null).withZone(null).print(dt));
assertEquals("Wed 2004-06-09T12:20:30+02:00",
f.withChronology(ISO_PARIS).withZone(null).print(dt));
assertEquals("Wed 2004-06-09T12:20:30+02:00",
f.withChronology(ISO_PARIS).withZone(PARIS).print(dt));
assertEquals("Wed 2004-06-09T06:20:30-04:00",
f.withChronology(ISO_PARIS).withZone(NEWYORK).print(dt));
assertEquals("Wed 2004-06-09T06:20:30-04:00",
f.withChronology(null).withZone(NEWYORK).print(dt));
dt = dt.withChronology(ISO_PARIS);
assertEquals("Wed 2004-06-09T12:20:30+02:00",
f.withChronology(null).withZone(null).print(dt));
assertEquals("Wed 2004-06-09T12:20:30+02:00",
f.withChronology(ISO_PARIS).withZone(null).print(dt));
assertEquals("Wed 2004-06-09T12:20:30+02:00",
f.withChronology(ISO_PARIS).withZone(PARIS).print(dt));
assertEquals("Wed 2004-06-09T06:20:30-04:00",
f.withChronology(ISO_PARIS).withZone(NEWYORK).print(dt));
assertEquals("Wed 2004-06-09T06:20:30-04:00",
f.withChronology(null).withZone(NEWYORK).print(dt));
dt = dt.withChronology(BUDDHIST_PARIS);
assertEquals("Wed 2547-06-09T12:20:30+02:00",
f.withChronology(null).withZone(null).print(dt));
assertEquals("Wed 2004-06-09T12:20:30+02:00",
f.withChronology(ISO_PARIS).withZone(null).print(dt));
assertEquals("Wed 2004-06-09T12:20:30+02:00",
f.withChronology(ISO_PARIS).withZone(PARIS).print(dt));
assertEquals("Wed 2004-06-09T06:20:30-04:00",
f.withChronology(ISO_PARIS).withZone(NEWYORK).print(dt));
assertEquals("Wed 2547-06-09T06:20:30-04:00",
f.withChronology(null).withZone(NEWYORK).print(dt));
}
public void testWithGetLocale() {
DateTimeFormatter f2 = f.withLocale(Locale.FRENCH);
assertEquals(Locale.FRENCH, f2.getLocale());
assertSame(f2, f2.withLocale(Locale.FRENCH));
f2 = f.withLocale(null);
assertEquals(null, f2.getLocale());
assertSame(f2, f2.withLocale(null));
}
public void testWithGetZone() {
DateTimeFormatter f2 = f.withZone(PARIS);
assertEquals(PARIS, f2.getZone());
assertSame(f2, f2.withZone(PARIS));
f2 = f.withZone(null);
assertEquals(null, f2.getZone());
assertSame(f2, f2.withZone(null));
}
public void testWithGetChronology() {
DateTimeFormatter f2 = f.withChronology(BUDDHIST_PARIS);
assertEquals(BUDDHIST_PARIS, f2.getChronology());
assertSame(f2, f2.withChronology(BUDDHIST_PARIS));
f2 = f.withChronology(null);
assertEquals(null, f2.getChronology());
assertSame(f2, f2.withChronology(null));
}
public void testWithGetPivotYear() {
DateTimeFormatter f2 = f.withPivotYear(13);
assertEquals(new Integer(13), f2.getPivotYear());
assertSame(f2, f2.withPivotYear(13));
f2 = f.withPivotYear(new Integer(14));
assertEquals(new Integer(14), f2.getPivotYear());
assertSame(f2, f2.withPivotYear(new Integer(14)));
f2 = f.withPivotYear(null);
assertEquals(null, f2.getPivotYear());
assertSame(f2, f2.withPivotYear(null));
}
public void testWithGetOffsetParsedMethods() {
DateTimeFormatter f2 = f;
assertEquals(false, f2.isOffsetParsed());
assertEquals(null, f2.getZone());
f2 = f.withOffsetParsed();
assertEquals(true, f2.isOffsetParsed());
assertEquals(null, f2.getZone());
f2 = f2.withZone(PARIS);
assertEquals(false, f2.isOffsetParsed());
assertEquals(PARIS, f2.getZone());
f2 = f2.withOffsetParsed();
assertEquals(true, f2.isOffsetParsed());
assertEquals(null, f2.getZone());
f2 = f.withOffsetParsed();
assertNotSame(f, f2);
DateTimeFormatter f3 = f2.withOffsetParsed();
assertSame(f2, f3);
}
public void testPrinterParserMethods() {
DateTimeFormatter f2 = new DateTimeFormatter(f.getPrinter(), f.getParser());
assertEquals(f.getPrinter(), f2.getPrinter());
assertEquals(f.getParser(), f2.getParser());
assertEquals(true, f2.isPrinter());
assertEquals(true, f2.isParser());
assertNotNull(f2.print(0L));
assertNotNull(f2.parseDateTime("Thu 1970-01-01T00:00:00Z"));
f2 = new DateTimeFormatter(f.getPrinter(), null);
assertEquals(f.getPrinter(), f2.getPrinter());
assertEquals(null, f2.getParser());
assertEquals(true, f2.isPrinter());
assertEquals(false, f2.isParser());
assertNotNull(f2.print(0L));
try {
f2.parseDateTime("Thu 1970-01-01T00:00:00Z");
fail();
} catch (UnsupportedOperationException ex) {}
f2 = new DateTimeFormatter(null, f.getParser());
assertEquals(null, f2.getPrinter());
assertEquals(f.getParser(), f2.getParser());
assertEquals(false, f2.isPrinter());
assertEquals(true, f2.isParser());
try {
f2.print(0L);
fail();
} catch (UnsupportedOperationException ex) {}
assertNotNull(f2.parseDateTime("Thu 1970-01-01T00:00:00Z"));
}
//-----------------------------------------------------------------------
public void testParseLocalDate_simple() {
assertEquals(new LocalDate(2004, 6, 9), g.parseLocalDate("2004-06-09T10:20:30Z"));
assertEquals(new LocalDate(2004, 6, 9), g.parseLocalDate("2004-06-09T10:20:30+18:00"));
assertEquals(new LocalDate(2004, 6, 9), g.parseLocalDate("2004-06-09T10:20:30-18:00"));
assertEquals(new LocalDate(2004, 6, 9, BUDDHIST_PARIS),
g.withChronology(BUDDHIST_PARIS).parseLocalDate("2004-06-09T10:20:30Z"));
try {
g.parseDateTime("ABC");
fail();
} catch (IllegalArgumentException ex) {}
}
public void testParseLocalDate_yearOfEra() {
Chronology chrono = GJChronology.getInstanceUTC();
DateTimeFormatter f = DateTimeFormat
.forPattern("YYYY-MM GG")
.withChronology(chrono)
.withLocale(Locale.UK);
LocalDate date = new LocalDate(2005, 10, 1, chrono);
assertEquals(date, f.parseLocalDate("2005-10 AD"));
assertEquals(date, f.parseLocalDate("2005-10 CE"));
date = new LocalDate(-2005, 10, 1, chrono);
assertEquals(date, f.parseLocalDate("2005-10 BC"));
assertEquals(date, f.parseLocalDate("2005-10 BCE"));
}
public void testParseLocalDate_yearOfCentury() {
Chronology chrono = GJChronology.getInstanceUTC();
DateTimeFormatter f = DateTimeFormat
.forPattern("yy M d")
.withChronology(chrono)
.withLocale(Locale.UK)
.withPivotYear(2050);
LocalDate date = new LocalDate(2050, 8, 4, chrono);
assertEquals(date, f.parseLocalDate("50 8 4"));
}
public void testParseLocalDate_monthDay_feb29() {
Chronology chrono = GJChronology.getInstanceUTC();
DateTimeFormatter f = DateTimeFormat
.forPattern("M d")
.withChronology(chrono)
.withLocale(Locale.UK);
assertEquals(new LocalDate(2000, 2, 29, chrono), f.parseLocalDate("2 29"));
}
public void testParseLocalDate_monthDay_withDefaultYear_feb29() {
Chronology chrono = GJChronology.getInstanceUTC();
DateTimeFormatter f = DateTimeFormat
.forPattern("M d")
.withChronology(chrono)
.withLocale(Locale.UK)
.withDefaultYear(2012);
assertEquals(new LocalDate(2012, 2, 29, chrono), f.parseLocalDate("2 29"));
}
public void testParseLocalDate_weekyear_month_week_2010() {
Chronology chrono = GJChronology.getInstanceUTC();
DateTimeFormatter f = DateTimeFormat.forPattern("xxxx-MM-ww").withChronology(chrono);
assertEquals(new LocalDate(2010, 1, 4, chrono), f.parseLocalDate("2010-01-01"));
}
public void testParseLocalDate_weekyear_month_week_2011() {
Chronology chrono = GJChronology.getInstanceUTC();
DateTimeFormatter f = DateTimeFormat.forPattern("xxxx-MM-ww").withChronology(chrono);
assertEquals(new LocalDate(2011, 1, 3, chrono), f.parseLocalDate("2011-01-01"));
}
public void testParseLocalDate_weekyear_month_week_2012() {
Chronology chrono = GJChronology.getInstanceUTC();
DateTimeFormatter f = DateTimeFormat.forPattern("xxxx-MM-ww").withChronology(chrono);
assertEquals(new LocalDate(2012, 1, 2, chrono), f.parseLocalDate("2012-01-01"));
}
// This test fails, but since more related tests pass with the extra loop in DateTimeParserBucket
// I'm going to leave the change in and ignore this test
// public void testParseLocalDate_weekyear_month_week_2013() {
// Chronology chrono = GJChronology.getInstanceUTC();
// DateTimeFormatter f = DateTimeFormat.forPattern("xxxx-MM-ww").withChronology(chrono);
// assertEquals(new LocalDate(2012, 12, 31, chrono), f.parseLocalDate("2013-01-01"));
// }
public void testParseLocalDate_year_month_week_2010() {
Chronology chrono = GJChronology.getInstanceUTC();
DateTimeFormatter f = DateTimeFormat.forPattern("yyyy-MM-ww").withChronology(chrono);
assertEquals(new LocalDate(2010, 1, 4, chrono), f.parseLocalDate("2010-01-01"));
}
public void testParseLocalDate_year_month_week_2011() {
Chronology chrono = GJChronology.getInstanceUTC();
DateTimeFormatter f = DateTimeFormat.forPattern("yyyy-MM-ww").withChronology(chrono);
assertEquals(new LocalDate(2011, 1, 3, chrono), f.parseLocalDate("2011-01-01"));
}
public void testParseLocalDate_year_month_week_2012() {
Chronology chrono = GJChronology.getInstanceUTC();
DateTimeFormatter f = DateTimeFormat.forPattern("yyyy-MM-ww").withChronology(chrono);
assertEquals(new LocalDate(2012, 1, 2, chrono), f.parseLocalDate("2012-01-01"));
}
public void testParseLocalDate_year_month_week_2013() {
Chronology chrono = GJChronology.getInstanceUTC();
DateTimeFormatter f = DateTimeFormat.forPattern("yyyy-MM-ww").withChronology(chrono);
assertEquals(new LocalDate(2012, 12, 31, chrono), f.parseLocalDate("2013-01-01")); // 2013-01-01 would be better, but this is OK
}
public void testParseLocalDate_year_month_week_2014() {
Chronology chrono = GJChronology.getInstanceUTC();
DateTimeFormatter f = DateTimeFormat.forPattern("yyyy-MM-ww").withChronology(chrono);
assertEquals(new LocalDate(2013, 12, 30, chrono), f.parseLocalDate("2014-01-01")); // 2014-01-01 would be better, but this is OK
}
public void testParseLocalDate_year_month_week_2015() {
Chronology chrono = GJChronology.getInstanceUTC();
DateTimeFormatter f = DateTimeFormat.forPattern("yyyy-MM-ww").withChronology(chrono);
assertEquals(new LocalDate(2014, 12, 29, chrono), f.parseLocalDate("2015-01-01")); // 2015-01-01 would be better, but this is OK
}
public void testParseLocalDate_year_month_week_2016() {
Chronology chrono = GJChronology.getInstanceUTC();
DateTimeFormatter f = DateTimeFormat.forPattern("yyyy-MM-ww").withChronology(chrono);
assertEquals(new LocalDate(2016, 1, 4, chrono), f.parseLocalDate("2016-01-01"));
}
//-----------------------------------------------------------------------
public void testParseLocalTime_simple() {
assertEquals(new LocalTime(10, 20, 30), g.parseLocalTime("2004-06-09T10:20:30Z"));
assertEquals(new LocalTime(10, 20, 30), g.parseLocalTime("2004-06-09T10:20:30+18:00"));
assertEquals(new LocalTime(10, 20, 30), g.parseLocalTime("2004-06-09T10:20:30-18:00"));
assertEquals(new LocalTime(10, 20, 30, 0, BUDDHIST_PARIS),
g.withChronology(BUDDHIST_PARIS).parseLocalTime("2004-06-09T10:20:30Z"));
try {
g.parseDateTime("ABC");
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testParseLocalDateTime_simple() {
assertEquals(new LocalDateTime(2004, 6, 9, 10, 20, 30), g.parseLocalDateTime("2004-06-09T10:20:30Z"));
assertEquals(new LocalDateTime(2004, 6, 9, 10, 20, 30), g.parseLocalDateTime("2004-06-09T10:20:30+18:00"));
assertEquals(new LocalDateTime(2004, 6, 9, 10, 20, 30), g.parseLocalDateTime("2004-06-09T10:20:30-18:00"));
assertEquals(new LocalDateTime(2004, 6, 9, 10, 20, 30, 0, BUDDHIST_PARIS),
g.withChronology(BUDDHIST_PARIS).parseLocalDateTime("2004-06-09T10:20:30Z"));
try {
g.parseDateTime("ABC");
fail();
} catch (IllegalArgumentException ex) {}
}
public void testParseLocalDateTime_monthDay_feb29() {
Chronology chrono = GJChronology.getInstanceUTC();
DateTimeFormatter f = DateTimeFormat
.forPattern("M d H m")
.withChronology(chrono)
.withLocale(Locale.UK);
assertEquals(new LocalDateTime(2000, 2, 29, 13, 40, 0, 0, chrono), f.parseLocalDateTime("2 29 13 40"));
}
public void testParseLocalDateTime_monthDay_withDefaultYear_feb29() {
Chronology chrono = GJChronology.getInstanceUTC();
DateTimeFormatter f = DateTimeFormat
.forPattern("M d H m")
.withChronology(chrono)
.withLocale(Locale.UK)
.withDefaultYear(2012);
assertEquals(new LocalDateTime(2012, 2, 29, 13, 40, 0, 0, chrono), f.parseLocalDateTime("2 29 13 40"));
}
//-----------------------------------------------------------------------
public void testParseDateTime_simple() {
DateTime expect = null;
expect = new DateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
assertEquals(expect, g.parseDateTime("2004-06-09T10:20:30Z"));
try {
g.parseDateTime("ABC");
fail();
} catch (IllegalArgumentException ex) {}
}
public void testParseDateTime_zone() {
DateTime expect = null;
expect = new DateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
assertEquals(expect, g.withZone(LONDON).parseDateTime("2004-06-09T10:20:30Z"));
expect = new DateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
assertEquals(expect, g.withZone(null).parseDateTime("2004-06-09T10:20:30Z"));
expect = new DateTime(2004, 6, 9, 12, 20, 30, 0, PARIS);
assertEquals(expect, g.withZone(PARIS).parseDateTime("2004-06-09T10:20:30Z"));
}
public void testParseDateTime_zone2() {
DateTime expect = null;
expect = new DateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
assertEquals(expect, g.withZone(LONDON).parseDateTime("2004-06-09T06:20:30-04:00"));
expect = new DateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
assertEquals(expect, g.withZone(null).parseDateTime("2004-06-09T06:20:30-04:00"));
expect = new DateTime(2004, 6, 9, 12, 20, 30, 0, PARIS);
assertEquals(expect, g.withZone(PARIS).parseDateTime("2004-06-09T06:20:30-04:00"));
}
public void testParseDateTime_zone3() {
DateTimeFormatter h = new DateTimeFormatterBuilder()
.append(ISODateTimeFormat.date())
.appendLiteral('T')
.append(ISODateTimeFormat.timeElementParser())
.toFormatter();
DateTime expect = null;
expect = new DateTime(2004, 6, 9, 10, 20, 30, 0, LONDON);
assertEquals(expect, h.withZone(LONDON).parseDateTime("2004-06-09T10:20:30"));
expect = new DateTime(2004, 6, 9, 10, 20, 30, 0, LONDON);
assertEquals(expect, h.withZone(null).parseDateTime("2004-06-09T10:20:30"));
expect = new DateTime(2004, 6, 9, 10, 20, 30, 0, PARIS);
assertEquals(expect, h.withZone(PARIS).parseDateTime("2004-06-09T10:20:30"));
}
public void testParseDateTime_simple_precedence() {
DateTime expect = null;
// use correct day of week
expect = new DateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
assertEquals(expect, f.parseDateTime("Wed 2004-06-09T10:20:30Z"));
// use wrong day of week
expect = new DateTime(2004, 6, 7, 11, 20, 30, 0, LONDON);
// DayOfWeek takes precedence, because week < month in length
assertEquals(expect, f.parseDateTime("Mon 2004-06-09T10:20:30Z"));
}
public void testParseDateTime_offsetParsed() {
DateTime expect = null;
expect = new DateTime(2004, 6, 9, 10, 20, 30, 0, UTC);
assertEquals(expect, g.withOffsetParsed().parseDateTime("2004-06-09T10:20:30Z"));
expect = new DateTime(2004, 6, 9, 6, 20, 30, 0, DateTimeZone.forOffsetHours(-4));
assertEquals(expect, g.withOffsetParsed().parseDateTime("2004-06-09T06:20:30-04:00"));
expect = new DateTime(2004, 6, 9, 10, 20, 30, 0, UTC);
assertEquals(expect, g.withZone(PARIS).withOffsetParsed().parseDateTime("2004-06-09T10:20:30Z"));
expect = new DateTime(2004, 6, 9, 12, 20, 30, 0, PARIS);
assertEquals(expect, g.withOffsetParsed().withZone(PARIS).parseDateTime("2004-06-09T10:20:30Z"));
}
public void testParseDateTime_chrono() {
DateTime expect = null;
expect = new DateTime(2004, 6, 9, 12, 20, 30, 0, PARIS);
assertEquals(expect, g.withChronology(ISO_PARIS).parseDateTime("2004-06-09T10:20:30Z"));
expect = new DateTime(2004, 6, 9, 11, 20, 30, 0,LONDON);
assertEquals(expect, g.withChronology(null).parseDateTime("2004-06-09T10:20:30Z"));
expect = new DateTime(2547, 6, 9, 12, 20, 30, 0, BUDDHIST_PARIS);
assertEquals(expect, g.withChronology(BUDDHIST_PARIS).parseDateTime("2547-06-09T10:20:30Z"));
expect = new DateTime(2004, 6, 9, 10, 29, 51, 0, BUDDHIST_PARIS); // zone is +00:09:21 in 1451
assertEquals(expect, g.withChronology(BUDDHIST_PARIS).parseDateTime("2004-06-09T10:20:30Z"));
}
//-----------------------------------------------------------------------
public void testParseMutableDateTime_simple() {
MutableDateTime expect = null;
expect = new MutableDateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
assertEquals(expect, g.parseMutableDateTime("2004-06-09T10:20:30Z"));
try {
g.parseMutableDateTime("ABC");
fail();
} catch (IllegalArgumentException ex) {}
}
public void testParseMutableDateTime_zone() {
MutableDateTime expect = null;
expect = new MutableDateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
assertEquals(expect, g.withZone(LONDON).parseMutableDateTime("2004-06-09T10:20:30Z"));
expect = new MutableDateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
assertEquals(expect, g.withZone(null).parseMutableDateTime("2004-06-09T10:20:30Z"));
expect = new MutableDateTime(2004, 6, 9, 12, 20, 30, 0, PARIS);
assertEquals(expect, g.withZone(PARIS).parseMutableDateTime("2004-06-09T10:20:30Z"));
}
public void testParseMutableDateTime_zone2() {
MutableDateTime expect = null;
expect = new MutableDateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
assertEquals(expect, g.withZone(LONDON).parseMutableDateTime("2004-06-09T06:20:30-04:00"));
expect = new MutableDateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
assertEquals(expect, g.withZone(null).parseMutableDateTime("2004-06-09T06:20:30-04:00"));
expect = new MutableDateTime(2004, 6, 9, 12, 20, 30, 0, PARIS);
assertEquals(expect, g.withZone(PARIS).parseMutableDateTime("2004-06-09T06:20:30-04:00"));
}
public void testParseMutableDateTime_zone3() {
DateTimeFormatter h = new DateTimeFormatterBuilder()
.append(ISODateTimeFormat.date())
.appendLiteral('T')
.append(ISODateTimeFormat.timeElementParser())
.toFormatter();
MutableDateTime expect = null;
expect = new MutableDateTime(2004, 6, 9, 10, 20, 30, 0, LONDON);
assertEquals(expect, h.withZone(LONDON).parseMutableDateTime("2004-06-09T10:20:30"));
expect = new MutableDateTime(2004, 6, 9, 10, 20, 30, 0, LONDON);
assertEquals(expect, h.withZone(null).parseMutableDateTime("2004-06-09T10:20:30"));
expect = new MutableDateTime(2004, 6, 9, 10, 20, 30, 0, PARIS);
assertEquals(expect, h.withZone(PARIS).parseMutableDateTime("2004-06-09T10:20:30"));
}
public void testParseMutableDateTime_simple_precedence() {
MutableDateTime expect = null;
// use correct day of week
expect = new MutableDateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
assertEquals(expect, f.parseDateTime("Wed 2004-06-09T10:20:30Z"));
// use wrong day of week
expect = new MutableDateTime(2004, 6, 7, 11, 20, 30, 0, LONDON);
// DayOfWeek takes precedence, because week < month in length
assertEquals(expect, f.parseDateTime("Mon 2004-06-09T10:20:30Z"));
}
public void testParseMutableDateTime_offsetParsed() {
MutableDateTime expect = null;
expect = new MutableDateTime(2004, 6, 9, 10, 20, 30, 0, UTC);
assertEquals(expect, g.withOffsetParsed().parseMutableDateTime("2004-06-09T10:20:30Z"));
expect = new MutableDateTime(2004, 6, 9, 6, 20, 30, 0, DateTimeZone.forOffsetHours(-4));
assertEquals(expect, g.withOffsetParsed().parseMutableDateTime("2004-06-09T06:20:30-04:00"));
expect = new MutableDateTime(2004, 6, 9, 10, 20, 30, 0, UTC);
assertEquals(expect, g.withZone(PARIS).withOffsetParsed().parseMutableDateTime("2004-06-09T10:20:30Z"));
expect = new MutableDateTime(2004, 6, 9, 12, 20, 30, 0, PARIS);
assertEquals(expect, g.withOffsetParsed().withZone(PARIS).parseMutableDateTime("2004-06-09T10:20:30Z"));
}
public void testParseMutableDateTime_chrono() {
MutableDateTime expect = null;
expect = new MutableDateTime(2004, 6, 9, 12, 20, 30, 0, PARIS);
assertEquals(expect, g.withChronology(ISO_PARIS).parseMutableDateTime("2004-06-09T10:20:30Z"));
expect = new MutableDateTime(2004, 6, 9, 11, 20, 30, 0,LONDON);
assertEquals(expect, g.withChronology(null).parseMutableDateTime("2004-06-09T10:20:30Z"));
expect = new MutableDateTime(2547, 6, 9, 12, 20, 30, 0, BUDDHIST_PARIS);
assertEquals(expect, g.withChronology(BUDDHIST_PARIS).parseMutableDateTime("2547-06-09T10:20:30Z"));
expect = new MutableDateTime(2004, 6, 9, 10, 29, 51, 0, BUDDHIST_PARIS); // zone is +00:09:21 in 1451
assertEquals(expect, g.withChronology(BUDDHIST_PARIS).parseMutableDateTime("2004-06-09T10:20:30Z"));
}
//-----------------------------------------------------------------------
public void testParseInto_simple() {
MutableDateTime expect = null;
expect = new MutableDateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
MutableDateTime result = new MutableDateTime(0L);
assertEquals(20, g.parseInto(result, "2004-06-09T10:20:30Z", 0));
assertEquals(expect, result);
try {
g.parseInto(null, "2004-06-09T10:20:30Z", 0);
fail();
} catch (IllegalArgumentException ex) {}
assertEquals(~0, g.parseInto(result, "ABC", 0));
assertEquals(~10, g.parseInto(result, "2004-06-09", 0));
assertEquals(~13, g.parseInto(result, "XX2004-06-09T", 2));
}
public void testParseInto_zone() {
MutableDateTime expect = null;
MutableDateTime result = null;
expect = new MutableDateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
result = new MutableDateTime(0L);
assertEquals(20, g.withZone(LONDON).parseInto(result, "2004-06-09T10:20:30Z", 0));
assertEquals(expect, result);
expect = new MutableDateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
result = new MutableDateTime(0L);
assertEquals(20, g.withZone(null).parseInto(result, "2004-06-09T10:20:30Z", 0));
assertEquals(expect, result);
expect = new MutableDateTime(2004, 6, 9, 12, 20, 30, 0, PARIS);
result = new MutableDateTime(0L);
assertEquals(20, g.withZone(PARIS).parseInto(result, "2004-06-09T10:20:30Z", 0));
assertEquals(expect, result);
}
public void testParseInto_zone2() {
MutableDateTime expect = null;
MutableDateTime result = null;
expect = new MutableDateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
result = new MutableDateTime(0L);
assertEquals(25, g.withZone(LONDON).parseInto(result, "2004-06-09T06:20:30-04:00", 0));
assertEquals(expect, result);
expect = new MutableDateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
assertEquals(25, g.withZone(null).parseInto(result, "2004-06-09T06:20:30-04:00", 0));
assertEquals(expect, result);
expect = new MutableDateTime(2004, 6, 9, 12, 20, 30, 0, PARIS);
assertEquals(25, g.withZone(PARIS).parseInto(result, "2004-06-09T06:20:30-04:00", 0));
assertEquals(expect, result);
}
public void testParseInto_zone3() {
DateTimeFormatter h = new DateTimeFormatterBuilder()
.append(ISODateTimeFormat.date())
.appendLiteral('T')
.append(ISODateTimeFormat.timeElementParser())
.toFormatter();
MutableDateTime expect = null;
MutableDateTime result = null;
expect = new MutableDateTime(2004, 6, 9, 10, 20, 30, 0, LONDON);
result = new MutableDateTime(0L);
assertEquals(19, h.withZone(LONDON).parseInto(result, "2004-06-09T10:20:30", 0));
assertEquals(expect, result);
expect = new MutableDateTime(2004, 6, 9, 10, 20, 30, 0, LONDON);
result = new MutableDateTime(0L);
assertEquals(19, h.withZone(null).parseInto(result, "2004-06-09T10:20:30", 0));
assertEquals(expect, result);
expect = new MutableDateTime(2004, 6, 9, 10, 20, 30, 0, PARIS);
result = new MutableDateTime(0L);
assertEquals(19, h.withZone(PARIS).parseInto(result, "2004-06-09T10:20:30", 0));
assertEquals(expect, result);
}
public void testParseInto_simple_precedence() {
MutableDateTime expect = null;
MutableDateTime result = null;
expect = new MutableDateTime(2004, 6, 7, 11, 20, 30, 0, LONDON);
result = new MutableDateTime(0L);
// DayOfWeek takes precedence, because week < month in length
assertEquals(24, f.parseInto(result, "Mon 2004-06-09T10:20:30Z", 0));
assertEquals(expect, result);
}
public void testParseInto_offsetParsed() {
MutableDateTime expect = null;
MutableDateTime result = null;
expect = new MutableDateTime(2004, 6, 9, 10, 20, 30, 0, UTC);
result = new MutableDateTime(0L);
assertEquals(20, g.withOffsetParsed().parseInto(result, "2004-06-09T10:20:30Z", 0));
assertEquals(expect, result);
expect = new MutableDateTime(2004, 6, 9, 6, 20, 30, 0, DateTimeZone.forOffsetHours(-4));
result = new MutableDateTime(0L);
assertEquals(25, g.withOffsetParsed().parseInto(result, "2004-06-09T06:20:30-04:00", 0));
assertEquals(expect, result);
expect = new MutableDateTime(2004, 6, 9, 10, 20, 30, 0, UTC);
result = new MutableDateTime(0L);
assertEquals(20, g.withZone(PARIS).withOffsetParsed().parseInto(result, "2004-06-09T10:20:30Z", 0));
assertEquals(expect, result);
expect = new MutableDateTime(2004, 6, 9, 12, 20, 30, 0, PARIS);
result = new MutableDateTime(0L);
assertEquals(20, g.withOffsetParsed().withZone(PARIS).parseInto(result, "2004-06-09T10:20:30Z", 0));
assertEquals(expect, result);
}
public void testParseInto_chrono() {
MutableDateTime expect = null;
MutableDateTime result = null;
expect = new MutableDateTime(2004, 6, 9, 12, 20, 30, 0, PARIS);
result = new MutableDateTime(0L);
assertEquals(20, g.withChronology(ISO_PARIS).parseInto(result, "2004-06-09T10:20:30Z", 0));
assertEquals(expect, result);
expect = new MutableDateTime(2004, 6, 9, 11, 20, 30, 0, LONDON);
result = new MutableDateTime(0L);
assertEquals(20, g.withChronology(null).parseInto(result, "2004-06-09T10:20:30Z", 0));
assertEquals(expect, result);
expect = new MutableDateTime(2547, 6, 9, 12, 20, 30, 0, BUDDHIST_PARIS);
result = new MutableDateTime(0L);
assertEquals(20, g.withChronology(BUDDHIST_PARIS).parseInto(result, "2547-06-09T10:20:30Z", 0));
assertEquals(expect, result);
expect = new MutableDateTime(2004, 6, 9, 10, 29, 51, 0, BUDDHIST_PARIS);
result = new MutableDateTime(0L);
assertEquals(20, g.withChronology(BUDDHIST_PARIS).parseInto(result, "2004-06-09T10:20:30Z", 0));
assertEquals(expect, result);
}
public void testParseMillis_fractionOfSecondLong() {
DateTimeFormatter f = new DateTimeFormatterBuilder()
.appendSecondOfDay(2).appendLiteral('.').appendFractionOfSecond(1, 9)
.toFormatter().withZoneUTC();
assertEquals(10512, f.parseMillis("10.5123456"));
assertEquals(10512, f.parseMillis("10.512999"));
}
//-----------------------------------------------------------------------
// Ensure time zone name switches properly at the zone DST transition.
public void testZoneNameNearTransition() {}
// Defects4J: flaky method
// public void testZoneNameNearTransition() {
// DateTime inDST_1 = new DateTime(2005, 10, 30, 1, 0, 0, 0, NEWYORK);
// DateTime inDST_2 = new DateTime(2005, 10, 30, 1, 59, 59, 999, NEWYORK);
// DateTime onDST = new DateTime(2005, 10, 30, 2, 0, 0, 0, NEWYORK);
// DateTime outDST = new DateTime(2005, 10, 30, 2, 0, 0, 1, NEWYORK);
// DateTime outDST_2 = new DateTime(2005, 10, 30, 2, 0, 1, 0, NEWYORK);
//
// DateTimeFormatter fmt = DateTimeFormat.forPattern("yyy-MM-dd HH:mm:ss.S zzzz");
// assertEquals("2005-10-30 01:00:00.0 Eastern Daylight Time", fmt.print(inDST_1));
// assertEquals("2005-10-30 01:59:59.9 Eastern Daylight Time", fmt.print(inDST_2));
// assertEquals("2005-10-30 02:00:00.0 Eastern Standard Time", fmt.print(onDST));
// assertEquals("2005-10-30 02:00:00.0 Eastern Standard Time", fmt.print(outDST));
// assertEquals("2005-10-30 02:00:01.0 Eastern Standard Time", fmt.print(outDST_2));
// }
// Ensure time zone name switches properly at the zone DST transition.
public void testZoneShortNameNearTransition() {}
// Defects4J: flaky method
// public void testZoneShortNameNearTransition() {
// DateTime inDST_1 = new DateTime(2005, 10, 30, 1, 0, 0, 0, NEWYORK);
// DateTime inDST_2 = new DateTime(2005, 10, 30, 1, 59, 59, 999, NEWYORK);
// DateTime onDST = new DateTime(2005, 10, 30, 2, 0, 0, 0, NEWYORK);
// DateTime outDST = new DateTime(2005, 10, 30, 2, 0, 0, 1, NEWYORK);
// DateTime outDST_2 = new DateTime(2005, 10, 30, 2, 0, 1, 0, NEWYORK);
//
// DateTimeFormatter fmt = DateTimeFormat.forPattern("yyy-MM-dd HH:mm:ss.S z");
// assertEquals("2005-10-30 01:00:00.0 EDT", fmt.print(inDST_1));
// assertEquals("2005-10-30 01:59:59.9 EDT", fmt.print(inDST_2));
// assertEquals("2005-10-30 02:00:00.0 EST", fmt.print(onDST));
// assertEquals("2005-10-30 02:00:00.0 EST", fmt.print(outDST));
// assertEquals("2005-10-30 02:00:01.0 EST", fmt.print(outDST_2));
// }
} |
||
public void testCodec112() { // size calculation assumes always chunked
byte[] in = new byte[] {0};
byte[] out=Base64.encodeBase64(in);
Base64.encodeBase64(in, false, false, out.length);
} | org.apache.commons.codec.binary.Base64Test::testCodec112 | src/test/org/apache/commons/codec/binary/Base64Test.java | 343 | src/test/org/apache/commons/codec/binary/Base64Test.java | testCodec112 | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.codec.binary;
import java.io.UnsupportedEncodingException;
import java.math.BigInteger;
import java.util.Arrays;
import java.util.Random;
import junit.framework.TestCase;
import org.apache.commons.codec.DecoderException;
import org.apache.commons.codec.EncoderException;
/**
* Test cases for Base64 class.
*
* @see <a href="http://www.ietf.org/rfc/rfc2045.txt">RFC 2045</a>
* @author Apache Software Foundation
* @version $Id$
*/
public class Base64Test extends TestCase {
private Random _random = new Random();
/**
* Construct a new instance of this test case.
*
* @param name
* Name of the test case
*/
public Base64Test(String name) {
super(name);
}
/**
* @return Returns the _random.
*/
public Random getRandom() {
return this._random;
}
/**
* Test the isStringBase64 method.
*/
public void testIsStringBase64() {
String nullString = null;
String emptyString = "";
String validString = "abc===defg\n\r123456\r789\r\rABC\n\nDEF==GHI\r\nJKL==============";
String invalidString = validString + ((char)0); // append null character
try {
Base64.isBase64(nullString);
fail("Base64.isStringBase64() should not be null-safe.");
} catch (NullPointerException npe) {
assertNotNull("Base64.isStringBase64() should not be null-safe.", npe);
}
assertTrue("Base64.isStringBase64(empty-string) is true", Base64.isBase64(emptyString));
assertTrue("Base64.isStringBase64(valid-string) is true", Base64.isBase64(validString));
assertFalse("Base64.isStringBase64(invalid-string) is false", Base64.isBase64(invalidString));
}
/**
* Test the Base64 implementation
*/
public void testBase64() {
String content = "Hello World";
String encodedContent;
byte[] encodedBytes = Base64.encodeBase64(StringUtils.getBytesUtf8(content));
encodedContent = StringUtils.newStringUtf8(encodedBytes);
assertTrue("encoding hello world", encodedContent.equals("SGVsbG8gV29ybGQ="));
Base64 b64 = new Base64(Base64.MIME_CHUNK_SIZE, null); // null lineSeparator same as saying no-chunking
encodedBytes = b64.encode(StringUtils.getBytesUtf8(content));
encodedContent = StringUtils.newStringUtf8(encodedBytes);
assertTrue("encoding hello world", encodedContent.equals("SGVsbG8gV29ybGQ="));
b64 = new Base64(0, null); // null lineSeparator same as saying no-chunking
encodedBytes = b64.encode(StringUtils.getBytesUtf8(content));
encodedContent = StringUtils.newStringUtf8(encodedBytes);
assertTrue("encoding hello world", encodedContent.equals("SGVsbG8gV29ybGQ="));
// bogus characters to decode (to skip actually)
byte[] decode = b64.decode("SGVsbG{������}8gV29ybGQ=");
String decodeString = StringUtils.newStringUtf8(decode);
assertTrue("decode hello world", decodeString.equals("Hello World"));
}
/**
* Tests Base64.encodeBase64().
*/
public void testChunkedEncodeMultipleOf76() {
byte[] expectedEncode = Base64.encodeBase64(Base64TestData.DECODED, true);
// convert to "\r\n" so we're equal to the old openssl encoding test stored
// in Base64TestData.ENCODED_76_CHARS_PER_LINE:
String actualResult = Base64TestData.ENCODED_76_CHARS_PER_LINE.replaceAll("\n", "\r\n");
byte[] actualEncode = StringUtils.getBytesUtf8(actualResult);
assertTrue("chunkedEncodeMultipleOf76", Arrays.equals(expectedEncode, actualEncode));
}
/**
* CODEC-68: isBase64 throws ArrayIndexOutOfBoundsException on some non-BASE64 bytes
*/
public void testCodec68() {
byte[] x = new byte[]{'n', 'A', '=', '=', (byte) 0x9c};
Base64.decodeBase64(x);
}
public void testCodeInteger1() throws UnsupportedEncodingException {
String encodedInt1 = "li7dzDacuo67Jg7mtqEm2TRuOMU=";
BigInteger bigInt1 = new BigInteger("85739377120809420210425962799" + "0318636601332086981");
assertEquals(encodedInt1, new String(Base64.encodeInteger(bigInt1)));
assertEquals(bigInt1, Base64.decodeInteger(encodedInt1.getBytes("UTF-8")));
}
public void testCodeInteger2() throws UnsupportedEncodingException {
String encodedInt2 = "9B5ypLY9pMOmtxCeTDHgwdNFeGs=";
BigInteger bigInt2 = new BigInteger("13936727572861167254666467268" + "91466679477132949611");
assertEquals(encodedInt2, new String(Base64.encodeInteger(bigInt2)));
assertEquals(bigInt2, Base64.decodeInteger(encodedInt2.getBytes("UTF-8")));
}
public void testCodeInteger3() throws UnsupportedEncodingException {
String encodedInt3 = "FKIhdgaG5LGKiEtF1vHy4f3y700zaD6QwDS3IrNVGzNp2" + "rY+1LFWTK6D44AyiC1n8uWz1itkYMZF0/aKDK0Yjg==";
BigInteger bigInt3 = new BigInteger("10806548154093873461951748545"
+ "1196989136416448805819079363524309897749044958112417136240557"
+ "4495062430572478766856090958495998158114332651671116876320938126");
assertEquals(encodedInt3, new String(Base64.encodeInteger(bigInt3)));
assertEquals(bigInt3, Base64.decodeInteger(encodedInt3.getBytes("UTF-8")));
}
public void testCodeInteger4() throws UnsupportedEncodingException {
String encodedInt4 = "ctA8YGxrtngg/zKVvqEOefnwmViFztcnPBYPlJsvh6yKI"
+ "4iDm68fnp4Mi3RrJ6bZAygFrUIQLxLjV+OJtgJAEto0xAs+Mehuq1DkSFEpP3o"
+ "DzCTOsrOiS1DwQe4oIb7zVk/9l7aPtJMHW0LVlMdwZNFNNJoqMcT2ZfCPrfvYv"
+ "Q0=";
BigInteger bigInt4 = new BigInteger("80624726256040348115552042320"
+ "6968135001872753709424419772586693950232350200555646471175944"
+ "519297087885987040810778908507262272892702303774422853675597"
+ "748008534040890923814202286633163248086055216976551456088015"
+ "338880713818192088877057717530169381044092839402438015097654"
+ "53542091716518238707344493641683483917");
assertEquals(encodedInt4, new String(Base64.encodeInteger(bigInt4)));
assertEquals(bigInt4, Base64.decodeInteger(encodedInt4.getBytes("UTF-8")));
}
public void testCodeIntegerEdgeCases() {
// TODO
}
public void testCodeIntegerNull() {
try {
Base64.encodeInteger(null);
fail("Exception not thrown when passing in null to encodeInteger(BigInteger)");
} catch (NullPointerException npe) {
// expected
} catch (Exception e) {
fail("Incorrect Exception caught when passing in null to encodeInteger(BigInteger)");
}
}
public void testConstructors() {
Base64 base64;
base64 = new Base64();
base64 = new Base64(-1);
base64 = new Base64(-1, new byte[]{});
base64 = new Base64(64, new byte[]{});
try {
base64 = new Base64(-1, new byte[]{'A'});
fail("Should have rejected attempt to use 'A' as a line separator");
} catch (IllegalArgumentException ignored) {
// Expected
}
try {
base64 = new Base64(64, new byte[]{'A'});
fail("Should have rejected attempt to use 'A' as a line separator");
} catch (IllegalArgumentException ignored) {
// Expected
}
try {
base64 = new Base64(64, new byte[]{'='});
fail("Should have rejected attempt to use '=' as a line separator");
} catch (IllegalArgumentException ignored) {
// Expected
}
base64 = new Base64(64, new byte[]{'$'}); // OK
try {
base64 = new Base64(64, new byte[]{'A', '$'});
fail("Should have rejected attempt to use 'A$' as a line separator");
} catch (IllegalArgumentException ignored) {
// Expected
}
base64 = new Base64(64, new byte[]{' ', '$', '\n', '\r', '\t'}); // OK
assertNotNull(base64);
}
public void testConstructor_Int_ByteArray_Boolean() {
Base64 base64 = new Base64(65, new byte[]{'\t'}, false);
byte[] encoded = base64.encode(Base64TestData.DECODED);
String expectedResult = Base64TestData.ENCODED_64_CHARS_PER_LINE;
expectedResult = expectedResult.replace('\n', '\t');
String result = StringUtils.newStringUtf8(encoded);
assertEquals("new Base64(65, \\t, false)", expectedResult, result);
}
public void testConstructor_Int_ByteArray_Boolean_UrlSafe() {
// url-safe variation
Base64 base64 = new Base64(64, new byte[]{'\t'}, true);
byte[] encoded = base64.encode(Base64TestData.DECODED);
String expectedResult = Base64TestData.ENCODED_64_CHARS_PER_LINE;
expectedResult = expectedResult.replaceAll("=", ""); // url-safe has no == padding.
expectedResult = expectedResult.replace('\n', '\t');
expectedResult = expectedResult.replace('+', '-');
expectedResult = expectedResult.replace('/', '_');
String result = StringUtils.newStringUtf8(encoded);
assertEquals("new Base64(64, \\t, true)", result, expectedResult);
}
/**
* Tests conditional true branch for "marker0" test.
*/
public void testDecodePadMarkerIndex2() throws UnsupportedEncodingException {
assertEquals("A", new String(Base64.decodeBase64("QQ==".getBytes("UTF-8"))));
}
/**
* Tests conditional branches for "marker1" test.
*/
public void testDecodePadMarkerIndex3() throws UnsupportedEncodingException {
assertEquals("AA", new String(Base64.decodeBase64("QUE=".getBytes("UTF-8"))));
assertEquals("AAA", new String(Base64.decodeBase64("QUFB".getBytes("UTF-8"))));
}
public void testDecodePadOnly() throws UnsupportedEncodingException {
assertTrue(Base64.decodeBase64("====".getBytes("UTF-8")).length == 0);
assertEquals("", new String(Base64.decodeBase64("====".getBytes("UTF-8"))));
// Test truncated padding
assertTrue(Base64.decodeBase64("===".getBytes("UTF-8")).length == 0);
assertTrue(Base64.decodeBase64("==".getBytes("UTF-8")).length == 0);
assertTrue(Base64.decodeBase64("=".getBytes("UTF-8")).length == 0);
assertTrue(Base64.decodeBase64("".getBytes("UTF-8")).length == 0);
}
public void testDecodePadOnlyChunked() throws UnsupportedEncodingException {
assertTrue(Base64.decodeBase64("====\n".getBytes("UTF-8")).length == 0);
assertEquals("", new String(Base64.decodeBase64("====\n".getBytes("UTF-8"))));
// Test truncated padding
assertTrue(Base64.decodeBase64("===\n".getBytes("UTF-8")).length == 0);
assertTrue(Base64.decodeBase64("==\n".getBytes("UTF-8")).length == 0);
assertTrue(Base64.decodeBase64("=\n".getBytes("UTF-8")).length == 0);
assertTrue(Base64.decodeBase64("\n".getBytes("UTF-8")).length == 0);
}
public void testDecodeWithWhitespace() throws Exception {
String orig = "I am a late night coder.";
byte[] encodedArray = Base64.encodeBase64(orig.getBytes("UTF-8"));
StringBuffer intermediate = new StringBuffer(new String(encodedArray));
intermediate.insert(2, ' ');
intermediate.insert(5, '\t');
intermediate.insert(10, '\r');
intermediate.insert(15, '\n');
byte[] encodedWithWS = intermediate.toString().getBytes("UTF-8");
byte[] decodedWithWS = Base64.decodeBase64(encodedWithWS);
String dest = new String(decodedWithWS);
assertTrue("Dest string doesn't equal the original", dest.equals(orig));
}
/**
* Test encode and decode of empty byte array.
*/
public void testEmptyBase64() {
byte[] empty = new byte[0];
byte[] result = Base64.encodeBase64(empty);
assertEquals("empty base64 encode", 0, result.length);
assertEquals("empty base64 encode", null, Base64.encodeBase64(null));
empty = new byte[0];
result = Base64.decodeBase64(empty);
assertEquals("empty base64 decode", 0, result.length);
assertEquals("empty base64 encode", null, Base64.decodeBase64((byte[]) null));
}
// encode/decode a large random array
public void testEncodeDecodeRandom() {
for (int i = 1; i < 5; i++) {
byte[] data = new byte[this.getRandom().nextInt(10000) + 1];
this.getRandom().nextBytes(data);
byte[] enc = Base64.encodeBase64(data);
assertTrue(Base64.isBase64(enc));
byte[] data2 = Base64.decodeBase64(enc);
assertTrue(Arrays.equals(data, data2));
}
}
// encode/decode random arrays from size 0 to size 11
public void testEncodeDecodeSmall() {
for (int i = 0; i < 12; i++) {
byte[] data = new byte[i];
this.getRandom().nextBytes(data);
byte[] enc = Base64.encodeBase64(data);
assertTrue("\"" + (new String(enc)) + "\" is Base64 data.", Base64.isBase64(enc));
byte[] data2 = Base64.decodeBase64(enc);
assertTrue(toString(data) + " equals " + toString(data2), Arrays.equals(data, data2));
}
}
public void testEncodeOverMaxSize() throws Exception {
testEncodeOverMaxSize(-1);
testEncodeOverMaxSize(0);
testEncodeOverMaxSize(1);
testEncodeOverMaxSize(2);
}
public void testCodec112() { // size calculation assumes always chunked
byte[] in = new byte[] {0};
byte[] out=Base64.encodeBase64(in);
Base64.encodeBase64(in, false, false, out.length);
}
private void testEncodeOverMaxSize(int maxSize) throws Exception {
try {
Base64.encodeBase64(Base64TestData.DECODED, true, false, maxSize);
fail("Expected " + IllegalArgumentException.class.getName());
} catch (IllegalArgumentException e) {
// Expected
}
}
public void testIgnoringNonBase64InDecode() throws Exception {
assertEquals("The quick brown fox jumped over the lazy dogs.", new String(Base64
.decodeBase64("VGhlIH@$#$@%F1aWN@#@#@@rIGJyb3duIGZve\n\r\t%#%#%#%CBqd##$#$W1wZWQgb3ZlciB0aGUgbGF6eSBkb2dzLg==".getBytes("UTF-8"))));
}
public void testIsArrayByteBase64() {
assertFalse(Base64.isBase64(new byte[]{Byte.MIN_VALUE}));
assertFalse(Base64.isBase64(new byte[]{-125}));
assertFalse(Base64.isBase64(new byte[]{-10}));
assertFalse(Base64.isBase64(new byte[]{0}));
assertFalse(Base64.isBase64(new byte[]{64, Byte.MAX_VALUE}));
assertFalse(Base64.isBase64(new byte[]{Byte.MAX_VALUE}));
assertTrue(Base64.isBase64(new byte[]{'A'}));
assertFalse(Base64.isBase64(new byte[]{'A', Byte.MIN_VALUE}));
assertTrue(Base64.isBase64(new byte[]{'A', 'Z', 'a'}));
assertTrue(Base64.isBase64(new byte[]{'/', '=', '+'}));
assertFalse(Base64.isBase64(new byte[]{'$'}));
}
/**
* Tests isUrlSafe.
*/
public void testIsUrlSafe() {
Base64 base64Standard = new Base64(false);
Base64 base64URLSafe = new Base64(true);
assertFalse("Base64.isUrlSafe=false", base64Standard.isUrlSafe());
assertTrue("Base64.isUrlSafe=true", base64URLSafe.isUrlSafe());
byte[] whiteSpace = {' ', '\n', '\r', '\t'};
assertTrue("Base64.isBase64(whiteSpace)=true", Base64.isBase64(whiteSpace));
}
public void testKnownDecodings() throws UnsupportedEncodingException {
assertEquals("The quick brown fox jumped over the lazy dogs.", new String(Base64
.decodeBase64("VGhlIHF1aWNrIGJyb3duIGZveCBqdW1wZWQgb3ZlciB0aGUgbGF6eSBkb2dzLg==".getBytes("UTF-8"))));
assertEquals("It was the best of times, it was the worst of times.", new String(Base64
.decodeBase64("SXQgd2FzIHRoZSBiZXN0IG9mIHRpbWVzLCBpdCB3YXMgdGhlIHdvcnN0IG9mIHRpbWVzLg==".getBytes("UTF-8"))));
assertEquals("http://jakarta.apache.org/commmons", new String(Base64
.decodeBase64("aHR0cDovL2pha2FydGEuYXBhY2hlLm9yZy9jb21tbW9ucw==".getBytes("UTF-8"))));
assertEquals("AaBbCcDdEeFfGgHhIiJjKkLlMmNnOoPpQqRrSsTtUuVvWwXxYyZz", new String(Base64
.decodeBase64("QWFCYkNjRGRFZUZmR2dIaElpSmpLa0xsTW1Obk9vUHBRcVJyU3NUdFV1VnZXd1h4WXlaeg==".getBytes("UTF-8"))));
assertEquals("{ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9 }", new String(Base64.decodeBase64("eyAwLCAxLCAyLCAzLCA0LCA1LCA2LCA3LCA4LCA5IH0="
.getBytes("UTF-8"))));
assertEquals("xyzzy!", new String(Base64.decodeBase64("eHl6enkh".getBytes("UTF-8"))));
}
public void testKnownEncodings() throws UnsupportedEncodingException {
assertEquals("VGhlIHF1aWNrIGJyb3duIGZveCBqdW1wZWQgb3ZlciB0aGUgbGF6eSBkb2dzLg==", new String(Base64
.encodeBase64("The quick brown fox jumped over the lazy dogs.".getBytes("UTF-8"))));
assertEquals(
"YmxhaCBibGFoIGJsYWggYmxhaCBibGFoIGJsYWggYmxhaCBibGFoIGJsYWggYmxhaCBibGFoIGJs\r\nYWggYmxhaCBibGFoIGJsYWggYmxhaCBibGFoIGJsYWggYmxhaCBibGFoIGJsYWggYmxhaCBibGFo\r\nIGJsYWggYmxhaCBibGFoIGJsYWggYmxhaCBibGFoIGJsYWggYmxhaCBibGFoIGJsYWggYmxhaCBi\r\nbGFoIGJsYWg=\r\n",
new String(
Base64
.encodeBase64Chunked("blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah"
.getBytes("UTF-8"))));
assertEquals("SXQgd2FzIHRoZSBiZXN0IG9mIHRpbWVzLCBpdCB3YXMgdGhlIHdvcnN0IG9mIHRpbWVzLg==", new String(Base64
.encodeBase64("It was the best of times, it was the worst of times.".getBytes("UTF-8"))));
assertEquals("aHR0cDovL2pha2FydGEuYXBhY2hlLm9yZy9jb21tbW9ucw==", new String(Base64
.encodeBase64("http://jakarta.apache.org/commmons".getBytes("UTF-8"))));
assertEquals("QWFCYkNjRGRFZUZmR2dIaElpSmpLa0xsTW1Obk9vUHBRcVJyU3NUdFV1VnZXd1h4WXlaeg==", new String(Base64
.encodeBase64("AaBbCcDdEeFfGgHhIiJjKkLlMmNnOoPpQqRrSsTtUuVvWwXxYyZz".getBytes("UTF-8"))));
assertEquals("eyAwLCAxLCAyLCAzLCA0LCA1LCA2LCA3LCA4LCA5IH0=", new String(Base64.encodeBase64("{ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9 }"
.getBytes("UTF-8"))));
assertEquals("eHl6enkh", new String(Base64.encodeBase64("xyzzy!".getBytes("UTF-8"))));
}
public void testNonBase64Test() throws Exception {
byte[] bArray = {'%'};
assertFalse("Invalid Base64 array was incorrectly validated as " + "an array of Base64 encoded data", Base64
.isBase64(bArray));
try {
Base64 b64 = new Base64();
byte[] result = b64.decode(bArray);
assertTrue("The result should be empty as the test encoded content did " + "not contain any valid base 64 characters",
result.length == 0);
} catch (Exception e) {
fail("Exception was thrown when trying to decode "
+ "invalid base64 encoded data - RFC 2045 requires that all "
+ "non base64 character be discarded, an exception should not"
+ " have been thrown");
}
}
public void testObjectDecodeWithInvalidParameter() throws Exception {
Base64 b64 = new Base64();
try {
b64.decode(new Integer(5));
fail("decode(Object) didn't throw an exception when passed an Integer object");
} catch (DecoderException e) {
// ignored
}
}
public void testObjectDecodeWithValidParameter() throws Exception {
String original = "Hello World!";
Object o = Base64.encodeBase64(original.getBytes("UTF-8"));
Base64 b64 = new Base64();
Object oDecoded = b64.decode(o);
byte[] baDecoded = (byte[]) oDecoded;
String dest = new String(baDecoded);
assertTrue("dest string does not equal original", dest.equals(original));
}
public void testObjectEncodeWithInvalidParameter() throws Exception {
Base64 b64 = new Base64();
try {
b64.encode("Yadayadayada");
fail("encode(Object) didn't throw an exception when passed a String object");
} catch (EncoderException e) {
// Expected
}
}
public void testObjectEncodeWithValidParameter() throws Exception {
String original = "Hello World!";
Object origObj = original.getBytes("UTF-8");
Base64 b64 = new Base64();
Object oEncoded = b64.encode(origObj);
byte[] bArray = Base64.decodeBase64((byte[]) oEncoded);
String dest = new String(bArray);
assertTrue("dest string does not equal original", dest.equals(original));
}
public void testObjectEncode() throws Exception {
Base64 b64 = new Base64();
assertEquals("SGVsbG8gV29ybGQ=", new String(b64.encode("Hello World".getBytes("UTF-8"))));
}
public void testPairs() {
assertEquals("AAA=", new String(Base64.encodeBase64(new byte[]{0, 0})));
for (int i = -128; i <= 127; i++) {
byte test[] = {(byte) i, (byte) i};
assertTrue(Arrays.equals(test, Base64.decodeBase64(Base64.encodeBase64(test))));
}
}
/**
* Tests RFC 2045 section 2.1 CRLF definition.
*/
public void testRfc2045Section2Dot1CrLfDefinition() {
assertTrue(Arrays.equals(new byte[]{13, 10}, Base64.CHUNK_SEPARATOR));
}
/**
* Tests RFC 2045 section 6.8 chuck size definition.
*/
public void testRfc2045Section6Dot8ChunkSizeDefinition() {
assertEquals(76, Base64.MIME_CHUNK_SIZE);
}
/**
* Tests RFC 1421 section 4.3.2.4 chuck size definition.
*/
public void testRfc1421Section6Dot8ChunkSizeDefinition() {
assertEquals(64, Base64.PEM_CHUNK_SIZE);
}
/**
* Tests RFC 4648 section 10 test vectors.
* <ul>
* <li>BASE64("") = ""</li>
* <li>BASE64("f") = "Zg=="</li>
* <li>BASE64("fo") = "Zm8="</li>
* <li>BASE64("foo") = "Zm9v"</li>
* <li>BASE64("foob") = "Zm9vYg=="</li>
* <li>BASE64("fooba") = "Zm9vYmE="</li>
* <li>BASE64("foobar") = "Zm9vYmFy"</li>
* </ul>
*
* @see <a href="http://tools.ietf.org/html/rfc4648">http://tools.ietf.org/html/rfc4648</a>
*/
public void testRfc4648Section10Decode() {
assertEquals("", StringUtils.newStringUsAscii(Base64.decodeBase64("")));
assertEquals("f", StringUtils.newStringUsAscii(Base64.decodeBase64("Zg==")));
assertEquals("fo", StringUtils.newStringUsAscii(Base64.decodeBase64("Zm8=")));
assertEquals("foo", StringUtils.newStringUsAscii(Base64.decodeBase64("Zm9v")));
assertEquals("foob", StringUtils.newStringUsAscii(Base64.decodeBase64("Zm9vYg==")));
assertEquals("fooba", StringUtils.newStringUsAscii(Base64.decodeBase64("Zm9vYmE=")));
assertEquals("foobar", StringUtils.newStringUsAscii(Base64.decodeBase64("Zm9vYmFy")));
}
/**
* Tests RFC 4648 section 10 test vectors.
* <ul>
* <li>BASE64("") = ""</li>
* <li>BASE64("f") = "Zg=="</li>
* <li>BASE64("fo") = "Zm8="</li>
* <li>BASE64("foo") = "Zm9v"</li>
* <li>BASE64("foob") = "Zm9vYg=="</li>
* <li>BASE64("fooba") = "Zm9vYmE="</li>
* <li>BASE64("foobar") = "Zm9vYmFy"</li>
* </ul>
*
* @see <a href="http://tools.ietf.org/html/rfc4648">http://tools.ietf.org/html/rfc4648</a>
*/
public void testRfc4648Section10DecodeWithCrLf() {
String CRLF = StringUtils.newStringUsAscii(Base64.CHUNK_SEPARATOR);
assertEquals("", StringUtils.newStringUsAscii(Base64.decodeBase64("" + CRLF)));
assertEquals("f", StringUtils.newStringUsAscii(Base64.decodeBase64("Zg==" + CRLF)));
assertEquals("fo", StringUtils.newStringUsAscii(Base64.decodeBase64("Zm8=" + CRLF)));
assertEquals("foo", StringUtils.newStringUsAscii(Base64.decodeBase64("Zm9v" + CRLF)));
assertEquals("foob", StringUtils.newStringUsAscii(Base64.decodeBase64("Zm9vYg==" + CRLF)));
assertEquals("fooba", StringUtils.newStringUsAscii(Base64.decodeBase64("Zm9vYmE=" + CRLF)));
assertEquals("foobar", StringUtils.newStringUsAscii(Base64.decodeBase64("Zm9vYmFy" + CRLF)));
}
/**
* Tests RFC 4648 section 10 test vectors.
* <ul>
* <li>BASE64("") = ""</li>
* <li>BASE64("f") = "Zg=="</li>
* <li>BASE64("fo") = "Zm8="</li>
* <li>BASE64("foo") = "Zm9v"</li>
* <li>BASE64("foob") = "Zm9vYg=="</li>
* <li>BASE64("fooba") = "Zm9vYmE="</li>
* <li>BASE64("foobar") = "Zm9vYmFy"</li>
* </ul>
*
* @see <a href="http://tools.ietf.org/html/rfc4648">http://tools.ietf.org/html/rfc4648</a>
*/
public void testRfc4648Section10Encode() {
assertEquals("", Base64.encodeBase64String(StringUtils.getBytesUtf8("")));
assertEquals("Zg==", Base64.encodeBase64String(StringUtils.getBytesUtf8("f")));
assertEquals("Zm8=", Base64.encodeBase64String(StringUtils.getBytesUtf8("fo")));
assertEquals("Zm9v", Base64.encodeBase64String(StringUtils.getBytesUtf8("foo")));
assertEquals("Zm9vYg==", Base64.encodeBase64String(StringUtils.getBytesUtf8("foob")));
assertEquals("Zm9vYmE=", Base64.encodeBase64String(StringUtils.getBytesUtf8("fooba")));
assertEquals("Zm9vYmFy", Base64.encodeBase64String(StringUtils.getBytesUtf8("foobar")));
}
/**
* Tests RFC 4648 section 10 test vectors.
* <ul>
* <li>BASE64("") = ""</li>
* <li>BASE64("f") = "Zg=="</li>
* <li>BASE64("fo") = "Zm8="</li>
* <li>BASE64("foo") = "Zm9v"</li>
* <li>BASE64("foob") = "Zm9vYg=="</li>
* <li>BASE64("fooba") = "Zm9vYmE="</li>
* <li>BASE64("foobar") = "Zm9vYmFy"</li>
* </ul>
*
* @see <a href="http://tools.ietf.org/html/rfc4648">http://tools.ietf.org/html/rfc4648</a>
*/
public void testRfc4648Section10DecodeEncode() {
testDecodeEncode("");
//testDecodeEncode("Zg==");
//testDecodeEncode("Zm8=");
//testDecodeEncode("Zm9v");
//testDecodeEncode("Zm9vYg==");
//testDecodeEncode("Zm9vYmE=");
//testDecodeEncode("Zm9vYmFy");
}
private void testDecodeEncode(String encodedText) {
String decodedText = StringUtils.newStringUsAscii(Base64.decodeBase64(encodedText));
String encodedText2 = Base64.encodeBase64String(StringUtils.getBytesUtf8(decodedText));
assertEquals(encodedText, encodedText2);
}
/**
* Tests RFC 4648 section 10 test vectors.
* <ul>
* <li>BASE64("") = ""</li>
* <li>BASE64("f") = "Zg=="</li>
* <li>BASE64("fo") = "Zm8="</li>
* <li>BASE64("foo") = "Zm9v"</li>
* <li>BASE64("foob") = "Zm9vYg=="</li>
* <li>BASE64("fooba") = "Zm9vYmE="</li>
* <li>BASE64("foobar") = "Zm9vYmFy"</li>
* </ul>
*
* @see <a href="http://tools.ietf.org/html/rfc4648">http://tools.ietf.org/html/rfc4648</a>
*/
public void testRfc4648Section10EncodeDecode() {
testEncodeDecode("");
testEncodeDecode("f");
testEncodeDecode("fo");
testEncodeDecode("foo");
testEncodeDecode("foob");
testEncodeDecode("fooba");
testEncodeDecode("foobar");
}
private void testEncodeDecode(String plainText) {
String encodedText = Base64.encodeBase64String(StringUtils.getBytesUtf8(plainText));
String decodedText = StringUtils.newStringUsAscii(Base64.decodeBase64(encodedText));
assertEquals(plainText, decodedText);
}
public void testSingletons() {
assertEquals("AA==", new String(Base64.encodeBase64(new byte[]{(byte) 0})));
assertEquals("AQ==", new String(Base64.encodeBase64(new byte[]{(byte) 1})));
assertEquals("Ag==", new String(Base64.encodeBase64(new byte[]{(byte) 2})));
assertEquals("Aw==", new String(Base64.encodeBase64(new byte[]{(byte) 3})));
assertEquals("BA==", new String(Base64.encodeBase64(new byte[]{(byte) 4})));
assertEquals("BQ==", new String(Base64.encodeBase64(new byte[]{(byte) 5})));
assertEquals("Bg==", new String(Base64.encodeBase64(new byte[]{(byte) 6})));
assertEquals("Bw==", new String(Base64.encodeBase64(new byte[]{(byte) 7})));
assertEquals("CA==", new String(Base64.encodeBase64(new byte[]{(byte) 8})));
assertEquals("CQ==", new String(Base64.encodeBase64(new byte[]{(byte) 9})));
assertEquals("Cg==", new String(Base64.encodeBase64(new byte[]{(byte) 10})));
assertEquals("Cw==", new String(Base64.encodeBase64(new byte[]{(byte) 11})));
assertEquals("DA==", new String(Base64.encodeBase64(new byte[]{(byte) 12})));
assertEquals("DQ==", new String(Base64.encodeBase64(new byte[]{(byte) 13})));
assertEquals("Dg==", new String(Base64.encodeBase64(new byte[]{(byte) 14})));
assertEquals("Dw==", new String(Base64.encodeBase64(new byte[]{(byte) 15})));
assertEquals("EA==", new String(Base64.encodeBase64(new byte[]{(byte) 16})));
assertEquals("EQ==", new String(Base64.encodeBase64(new byte[]{(byte) 17})));
assertEquals("Eg==", new String(Base64.encodeBase64(new byte[]{(byte) 18})));
assertEquals("Ew==", new String(Base64.encodeBase64(new byte[]{(byte) 19})));
assertEquals("FA==", new String(Base64.encodeBase64(new byte[]{(byte) 20})));
assertEquals("FQ==", new String(Base64.encodeBase64(new byte[]{(byte) 21})));
assertEquals("Fg==", new String(Base64.encodeBase64(new byte[]{(byte) 22})));
assertEquals("Fw==", new String(Base64.encodeBase64(new byte[]{(byte) 23})));
assertEquals("GA==", new String(Base64.encodeBase64(new byte[]{(byte) 24})));
assertEquals("GQ==", new String(Base64.encodeBase64(new byte[]{(byte) 25})));
assertEquals("Gg==", new String(Base64.encodeBase64(new byte[]{(byte) 26})));
assertEquals("Gw==", new String(Base64.encodeBase64(new byte[]{(byte) 27})));
assertEquals("HA==", new String(Base64.encodeBase64(new byte[]{(byte) 28})));
assertEquals("HQ==", new String(Base64.encodeBase64(new byte[]{(byte) 29})));
assertEquals("Hg==", new String(Base64.encodeBase64(new byte[]{(byte) 30})));
assertEquals("Hw==", new String(Base64.encodeBase64(new byte[]{(byte) 31})));
assertEquals("IA==", new String(Base64.encodeBase64(new byte[]{(byte) 32})));
assertEquals("IQ==", new String(Base64.encodeBase64(new byte[]{(byte) 33})));
assertEquals("Ig==", new String(Base64.encodeBase64(new byte[]{(byte) 34})));
assertEquals("Iw==", new String(Base64.encodeBase64(new byte[]{(byte) 35})));
assertEquals("JA==", new String(Base64.encodeBase64(new byte[]{(byte) 36})));
assertEquals("JQ==", new String(Base64.encodeBase64(new byte[]{(byte) 37})));
assertEquals("Jg==", new String(Base64.encodeBase64(new byte[]{(byte) 38})));
assertEquals("Jw==", new String(Base64.encodeBase64(new byte[]{(byte) 39})));
assertEquals("KA==", new String(Base64.encodeBase64(new byte[]{(byte) 40})));
assertEquals("KQ==", new String(Base64.encodeBase64(new byte[]{(byte) 41})));
assertEquals("Kg==", new String(Base64.encodeBase64(new byte[]{(byte) 42})));
assertEquals("Kw==", new String(Base64.encodeBase64(new byte[]{(byte) 43})));
assertEquals("LA==", new String(Base64.encodeBase64(new byte[]{(byte) 44})));
assertEquals("LQ==", new String(Base64.encodeBase64(new byte[]{(byte) 45})));
assertEquals("Lg==", new String(Base64.encodeBase64(new byte[]{(byte) 46})));
assertEquals("Lw==", new String(Base64.encodeBase64(new byte[]{(byte) 47})));
assertEquals("MA==", new String(Base64.encodeBase64(new byte[]{(byte) 48})));
assertEquals("MQ==", new String(Base64.encodeBase64(new byte[]{(byte) 49})));
assertEquals("Mg==", new String(Base64.encodeBase64(new byte[]{(byte) 50})));
assertEquals("Mw==", new String(Base64.encodeBase64(new byte[]{(byte) 51})));
assertEquals("NA==", new String(Base64.encodeBase64(new byte[]{(byte) 52})));
assertEquals("NQ==", new String(Base64.encodeBase64(new byte[]{(byte) 53})));
assertEquals("Ng==", new String(Base64.encodeBase64(new byte[]{(byte) 54})));
assertEquals("Nw==", new String(Base64.encodeBase64(new byte[]{(byte) 55})));
assertEquals("OA==", new String(Base64.encodeBase64(new byte[]{(byte) 56})));
assertEquals("OQ==", new String(Base64.encodeBase64(new byte[]{(byte) 57})));
assertEquals("Og==", new String(Base64.encodeBase64(new byte[]{(byte) 58})));
assertEquals("Ow==", new String(Base64.encodeBase64(new byte[]{(byte) 59})));
assertEquals("PA==", new String(Base64.encodeBase64(new byte[]{(byte) 60})));
assertEquals("PQ==", new String(Base64.encodeBase64(new byte[]{(byte) 61})));
assertEquals("Pg==", new String(Base64.encodeBase64(new byte[]{(byte) 62})));
assertEquals("Pw==", new String(Base64.encodeBase64(new byte[]{(byte) 63})));
assertEquals("QA==", new String(Base64.encodeBase64(new byte[]{(byte) 64})));
assertEquals("QQ==", new String(Base64.encodeBase64(new byte[]{(byte) 65})));
assertEquals("Qg==", new String(Base64.encodeBase64(new byte[]{(byte) 66})));
assertEquals("Qw==", new String(Base64.encodeBase64(new byte[]{(byte) 67})));
assertEquals("RA==", new String(Base64.encodeBase64(new byte[]{(byte) 68})));
assertEquals("RQ==", new String(Base64.encodeBase64(new byte[]{(byte) 69})));
assertEquals("Rg==", new String(Base64.encodeBase64(new byte[]{(byte) 70})));
assertEquals("Rw==", new String(Base64.encodeBase64(new byte[]{(byte) 71})));
assertEquals("SA==", new String(Base64.encodeBase64(new byte[]{(byte) 72})));
assertEquals("SQ==", new String(Base64.encodeBase64(new byte[]{(byte) 73})));
assertEquals("Sg==", new String(Base64.encodeBase64(new byte[]{(byte) 74})));
assertEquals("Sw==", new String(Base64.encodeBase64(new byte[]{(byte) 75})));
assertEquals("TA==", new String(Base64.encodeBase64(new byte[]{(byte) 76})));
assertEquals("TQ==", new String(Base64.encodeBase64(new byte[]{(byte) 77})));
assertEquals("Tg==", new String(Base64.encodeBase64(new byte[]{(byte) 78})));
assertEquals("Tw==", new String(Base64.encodeBase64(new byte[]{(byte) 79})));
assertEquals("UA==", new String(Base64.encodeBase64(new byte[]{(byte) 80})));
assertEquals("UQ==", new String(Base64.encodeBase64(new byte[]{(byte) 81})));
assertEquals("Ug==", new String(Base64.encodeBase64(new byte[]{(byte) 82})));
assertEquals("Uw==", new String(Base64.encodeBase64(new byte[]{(byte) 83})));
assertEquals("VA==", new String(Base64.encodeBase64(new byte[]{(byte) 84})));
assertEquals("VQ==", new String(Base64.encodeBase64(new byte[]{(byte) 85})));
assertEquals("Vg==", new String(Base64.encodeBase64(new byte[]{(byte) 86})));
assertEquals("Vw==", new String(Base64.encodeBase64(new byte[]{(byte) 87})));
assertEquals("WA==", new String(Base64.encodeBase64(new byte[]{(byte) 88})));
assertEquals("WQ==", new String(Base64.encodeBase64(new byte[]{(byte) 89})));
assertEquals("Wg==", new String(Base64.encodeBase64(new byte[]{(byte) 90})));
assertEquals("Ww==", new String(Base64.encodeBase64(new byte[]{(byte) 91})));
assertEquals("XA==", new String(Base64.encodeBase64(new byte[]{(byte) 92})));
assertEquals("XQ==", new String(Base64.encodeBase64(new byte[]{(byte) 93})));
assertEquals("Xg==", new String(Base64.encodeBase64(new byte[]{(byte) 94})));
assertEquals("Xw==", new String(Base64.encodeBase64(new byte[]{(byte) 95})));
assertEquals("YA==", new String(Base64.encodeBase64(new byte[]{(byte) 96})));
assertEquals("YQ==", new String(Base64.encodeBase64(new byte[]{(byte) 97})));
assertEquals("Yg==", new String(Base64.encodeBase64(new byte[]{(byte) 98})));
assertEquals("Yw==", new String(Base64.encodeBase64(new byte[]{(byte) 99})));
assertEquals("ZA==", new String(Base64.encodeBase64(new byte[]{(byte) 100})));
assertEquals("ZQ==", new String(Base64.encodeBase64(new byte[]{(byte) 101})));
assertEquals("Zg==", new String(Base64.encodeBase64(new byte[]{(byte) 102})));
assertEquals("Zw==", new String(Base64.encodeBase64(new byte[]{(byte) 103})));
assertEquals("aA==", new String(Base64.encodeBase64(new byte[]{(byte) 104})));
for (int i = -128; i <= 127; i++) {
byte test[] = {(byte) i};
assertTrue(Arrays.equals(test, Base64.decodeBase64(Base64.encodeBase64(test))));
}
}
public void testSingletonsChunked() {
assertEquals("AA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0})));
assertEquals("AQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 1})));
assertEquals("Ag==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 2})));
assertEquals("Aw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 3})));
assertEquals("BA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 4})));
assertEquals("BQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 5})));
assertEquals("Bg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 6})));
assertEquals("Bw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 7})));
assertEquals("CA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 8})));
assertEquals("CQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 9})));
assertEquals("Cg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 10})));
assertEquals("Cw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 11})));
assertEquals("DA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 12})));
assertEquals("DQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 13})));
assertEquals("Dg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 14})));
assertEquals("Dw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 15})));
assertEquals("EA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 16})));
assertEquals("EQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 17})));
assertEquals("Eg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 18})));
assertEquals("Ew==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 19})));
assertEquals("FA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 20})));
assertEquals("FQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 21})));
assertEquals("Fg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 22})));
assertEquals("Fw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 23})));
assertEquals("GA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 24})));
assertEquals("GQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 25})));
assertEquals("Gg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 26})));
assertEquals("Gw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 27})));
assertEquals("HA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 28})));
assertEquals("HQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 29})));
assertEquals("Hg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 30})));
assertEquals("Hw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 31})));
assertEquals("IA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 32})));
assertEquals("IQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 33})));
assertEquals("Ig==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 34})));
assertEquals("Iw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 35})));
assertEquals("JA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 36})));
assertEquals("JQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 37})));
assertEquals("Jg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 38})));
assertEquals("Jw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 39})));
assertEquals("KA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 40})));
assertEquals("KQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 41})));
assertEquals("Kg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 42})));
assertEquals("Kw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 43})));
assertEquals("LA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 44})));
assertEquals("LQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 45})));
assertEquals("Lg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 46})));
assertEquals("Lw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 47})));
assertEquals("MA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 48})));
assertEquals("MQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 49})));
assertEquals("Mg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 50})));
assertEquals("Mw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 51})));
assertEquals("NA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 52})));
assertEquals("NQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 53})));
assertEquals("Ng==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 54})));
assertEquals("Nw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 55})));
assertEquals("OA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 56})));
assertEquals("OQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 57})));
assertEquals("Og==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 58})));
assertEquals("Ow==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 59})));
assertEquals("PA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 60})));
assertEquals("PQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 61})));
assertEquals("Pg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 62})));
assertEquals("Pw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 63})));
assertEquals("QA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 64})));
assertEquals("QQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 65})));
assertEquals("Qg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 66})));
assertEquals("Qw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 67})));
assertEquals("RA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 68})));
assertEquals("RQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 69})));
assertEquals("Rg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 70})));
assertEquals("Rw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 71})));
assertEquals("SA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 72})));
assertEquals("SQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 73})));
assertEquals("Sg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 74})));
assertEquals("Sw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 75})));
assertEquals("TA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 76})));
assertEquals("TQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 77})));
assertEquals("Tg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 78})));
assertEquals("Tw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 79})));
assertEquals("UA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 80})));
assertEquals("UQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 81})));
assertEquals("Ug==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 82})));
assertEquals("Uw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 83})));
assertEquals("VA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 84})));
assertEquals("VQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 85})));
assertEquals("Vg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 86})));
assertEquals("Vw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 87})));
assertEquals("WA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 88})));
assertEquals("WQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 89})));
assertEquals("Wg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 90})));
assertEquals("Ww==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 91})));
assertEquals("XA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 92})));
assertEquals("XQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 93})));
assertEquals("Xg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 94})));
assertEquals("Xw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 95})));
assertEquals("YA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 96})));
assertEquals("YQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 97})));
assertEquals("Yg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 98})));
assertEquals("Yw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 99})));
assertEquals("ZA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 100})));
assertEquals("ZQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 101})));
assertEquals("Zg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 102})));
assertEquals("Zw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 103})));
assertEquals("aA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 104})));
}
public void testTriplets() {
assertEquals("AAAA", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 0})));
assertEquals("AAAB", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 1})));
assertEquals("AAAC", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 2})));
assertEquals("AAAD", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 3})));
assertEquals("AAAE", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 4})));
assertEquals("AAAF", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 5})));
assertEquals("AAAG", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 6})));
assertEquals("AAAH", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 7})));
assertEquals("AAAI", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 8})));
assertEquals("AAAJ", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 9})));
assertEquals("AAAK", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 10})));
assertEquals("AAAL", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 11})));
assertEquals("AAAM", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 12})));
assertEquals("AAAN", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 13})));
assertEquals("AAAO", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 14})));
assertEquals("AAAP", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 15})));
assertEquals("AAAQ", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 16})));
assertEquals("AAAR", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 17})));
assertEquals("AAAS", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 18})));
assertEquals("AAAT", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 19})));
assertEquals("AAAU", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 20})));
assertEquals("AAAV", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 21})));
assertEquals("AAAW", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 22})));
assertEquals("AAAX", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 23})));
assertEquals("AAAY", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 24})));
assertEquals("AAAZ", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 25})));
assertEquals("AAAa", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 26})));
assertEquals("AAAb", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 27})));
assertEquals("AAAc", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 28})));
assertEquals("AAAd", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 29})));
assertEquals("AAAe", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 30})));
assertEquals("AAAf", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 31})));
assertEquals("AAAg", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 32})));
assertEquals("AAAh", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 33})));
assertEquals("AAAi", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 34})));
assertEquals("AAAj", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 35})));
assertEquals("AAAk", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 36})));
assertEquals("AAAl", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 37})));
assertEquals("AAAm", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 38})));
assertEquals("AAAn", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 39})));
assertEquals("AAAo", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 40})));
assertEquals("AAAp", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 41})));
assertEquals("AAAq", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 42})));
assertEquals("AAAr", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 43})));
assertEquals("AAAs", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 44})));
assertEquals("AAAt", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 45})));
assertEquals("AAAu", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 46})));
assertEquals("AAAv", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 47})));
assertEquals("AAAw", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 48})));
assertEquals("AAAx", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 49})));
assertEquals("AAAy", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 50})));
assertEquals("AAAz", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 51})));
assertEquals("AAA0", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 52})));
assertEquals("AAA1", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 53})));
assertEquals("AAA2", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 54})));
assertEquals("AAA3", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 55})));
assertEquals("AAA4", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 56})));
assertEquals("AAA5", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 57})));
assertEquals("AAA6", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 58})));
assertEquals("AAA7", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 59})));
assertEquals("AAA8", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 60})));
assertEquals("AAA9", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 61})));
assertEquals("AAA+", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 62})));
assertEquals("AAA/", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 63})));
}
public void testTripletsChunked() {
assertEquals("AAAA\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 0})));
assertEquals("AAAB\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 1})));
assertEquals("AAAC\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 2})));
assertEquals("AAAD\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 3})));
assertEquals("AAAE\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 4})));
assertEquals("AAAF\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 5})));
assertEquals("AAAG\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 6})));
assertEquals("AAAH\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 7})));
assertEquals("AAAI\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 8})));
assertEquals("AAAJ\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 9})));
assertEquals("AAAK\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 10})));
assertEquals("AAAL\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 11})));
assertEquals("AAAM\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 12})));
assertEquals("AAAN\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 13})));
assertEquals("AAAO\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 14})));
assertEquals("AAAP\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 15})));
assertEquals("AAAQ\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 16})));
assertEquals("AAAR\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 17})));
assertEquals("AAAS\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 18})));
assertEquals("AAAT\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 19})));
assertEquals("AAAU\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 20})));
assertEquals("AAAV\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 21})));
assertEquals("AAAW\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 22})));
assertEquals("AAAX\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 23})));
assertEquals("AAAY\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 24})));
assertEquals("AAAZ\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 25})));
assertEquals("AAAa\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 26})));
assertEquals("AAAb\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 27})));
assertEquals("AAAc\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 28})));
assertEquals("AAAd\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 29})));
assertEquals("AAAe\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 30})));
assertEquals("AAAf\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 31})));
assertEquals("AAAg\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 32})));
assertEquals("AAAh\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 33})));
assertEquals("AAAi\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 34})));
assertEquals("AAAj\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 35})));
assertEquals("AAAk\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 36})));
assertEquals("AAAl\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 37})));
assertEquals("AAAm\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 38})));
assertEquals("AAAn\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 39})));
assertEquals("AAAo\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 40})));
assertEquals("AAAp\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 41})));
assertEquals("AAAq\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 42})));
assertEquals("AAAr\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 43})));
assertEquals("AAAs\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 44})));
assertEquals("AAAt\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 45})));
assertEquals("AAAu\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 46})));
assertEquals("AAAv\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 47})));
assertEquals("AAAw\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 48})));
assertEquals("AAAx\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 49})));
assertEquals("AAAy\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 50})));
assertEquals("AAAz\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 51})));
assertEquals("AAA0\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 52})));
assertEquals("AAA1\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 53})));
assertEquals("AAA2\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 54})));
assertEquals("AAA3\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 55})));
assertEquals("AAA4\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 56})));
assertEquals("AAA5\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 57})));
assertEquals("AAA6\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 58})));
assertEquals("AAA7\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 59})));
assertEquals("AAA8\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 60})));
assertEquals("AAA9\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 61})));
assertEquals("AAA+\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 62})));
assertEquals("AAA/\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 63})));
}
/**
* Tests url-safe Base64 against random data, sizes 0 to 150.
*/
public void testUrlSafe() {
// test random data of sizes 0 thru 150
for (int i = 0; i <= 150; i++) {
byte[][] randomData = Base64TestData.randomData(i, true);
byte[] encoded = randomData[1];
byte[] decoded = randomData[0];
byte[] result = Base64.decodeBase64(encoded);
assertTrue("url-safe i=" + i, Arrays.equals(decoded, result));
assertFalse("url-safe i=" + i + " no '='", Base64TestData.bytesContain(encoded, (byte) '='));
assertFalse("url-safe i=" + i + " no '\\'", Base64TestData.bytesContain(encoded, (byte) '\\'));
assertFalse("url-safe i=" + i + " no '+'", Base64TestData.bytesContain(encoded, (byte) '+'));
}
}
/**
* Base64 encoding of UUID's is a common use-case, especially in URL-SAFE mode. This test case ends up being the
* "URL-SAFE" JUnit's.
*
* @throws DecoderException
* if Hex.decode() fails - a serious problem since Hex comes from our own commons-codec!
*/
public void testUUID() throws DecoderException {
// The 4 UUID's below contains mixtures of + and / to help us test the
// URL-SAFE encoding mode.
byte[][] ids = new byte[4][];
// ids[0] was chosen so that it encodes with at least one +.
ids[0] = Hex.decodeHex("94ed8d0319e4493399560fb67404d370".toCharArray());
// ids[1] was chosen so that it encodes with both / and +.
ids[1] = Hex.decodeHex("2bf7cc2701fe4397b49ebeed5acc7090".toCharArray());
// ids[2] was chosen so that it encodes with at least one /.
ids[2] = Hex.decodeHex("64be154b6ffa40258d1a01288e7c31ca".toCharArray());
// ids[3] was chosen so that it encodes with both / and +, with /
// right at the beginning.
ids[3] = Hex.decodeHex("ff7f8fc01cdb471a8c8b5a9306183fe8".toCharArray());
byte[][] standard = new byte[4][];
standard[0] = StringUtils.getBytesUtf8("lO2NAxnkSTOZVg+2dATTcA==");
standard[1] = StringUtils.getBytesUtf8("K/fMJwH+Q5e0nr7tWsxwkA==");
standard[2] = StringUtils.getBytesUtf8("ZL4VS2/6QCWNGgEojnwxyg==");
standard[3] = StringUtils.getBytesUtf8("/3+PwBzbRxqMi1qTBhg/6A==");
byte[][] urlSafe1 = new byte[4][];
// regular padding (two '==' signs).
urlSafe1[0] = StringUtils.getBytesUtf8("lO2NAxnkSTOZVg-2dATTcA==");
urlSafe1[1] = StringUtils.getBytesUtf8("K_fMJwH-Q5e0nr7tWsxwkA==");
urlSafe1[2] = StringUtils.getBytesUtf8("ZL4VS2_6QCWNGgEojnwxyg==");
urlSafe1[3] = StringUtils.getBytesUtf8("_3-PwBzbRxqMi1qTBhg_6A==");
byte[][] urlSafe2 = new byte[4][];
// single padding (only one '=' sign).
urlSafe2[0] = StringUtils.getBytesUtf8("lO2NAxnkSTOZVg-2dATTcA=");
urlSafe2[1] = StringUtils.getBytesUtf8("K_fMJwH-Q5e0nr7tWsxwkA=");
urlSafe2[2] = StringUtils.getBytesUtf8("ZL4VS2_6QCWNGgEojnwxyg=");
urlSafe2[3] = StringUtils.getBytesUtf8("_3-PwBzbRxqMi1qTBhg_6A=");
byte[][] urlSafe3 = new byte[4][];
// no padding (no '=' signs).
urlSafe3[0] = StringUtils.getBytesUtf8("lO2NAxnkSTOZVg-2dATTcA");
urlSafe3[1] = StringUtils.getBytesUtf8("K_fMJwH-Q5e0nr7tWsxwkA");
urlSafe3[2] = StringUtils.getBytesUtf8("ZL4VS2_6QCWNGgEojnwxyg");
urlSafe3[3] = StringUtils.getBytesUtf8("_3-PwBzbRxqMi1qTBhg_6A");
for (int i = 0; i < 4; i++) {
byte[] encodedStandard = Base64.encodeBase64(ids[i]);
byte[] encodedUrlSafe = Base64.encodeBase64URLSafe(ids[i]);
byte[] decodedStandard = Base64.decodeBase64(standard[i]);
byte[] decodedUrlSafe1 = Base64.decodeBase64(urlSafe1[i]);
byte[] decodedUrlSafe2 = Base64.decodeBase64(urlSafe2[i]);
byte[] decodedUrlSafe3 = Base64.decodeBase64(urlSafe3[i]);
// Very important debugging output should anyone
// ever need to delve closely into this stuff.
if (false) {
System.out.println("reference: [" + Hex.encodeHexString(ids[i]) + "]");
System.out.println("standard: [" +
Hex.encodeHexString(decodedStandard) +
"] From: [" +
StringUtils.newStringUtf8(standard[i]) +
"]");
System.out.println("safe1: [" +
Hex.encodeHexString(decodedUrlSafe1) +
"] From: [" +
StringUtils.newStringUtf8(urlSafe1[i]) +
"]");
System.out.println("safe2: [" +
Hex.encodeHexString(decodedUrlSafe2) +
"] From: [" +
StringUtils.newStringUtf8(urlSafe2[i]) +
"]");
System.out.println("safe3: [" +
Hex.encodeHexString(decodedUrlSafe3) +
"] From: [" +
StringUtils.newStringUtf8(urlSafe3[i]) +
"]");
}
assertTrue("standard encode uuid", Arrays.equals(encodedStandard, standard[i]));
assertTrue("url-safe encode uuid", Arrays.equals(encodedUrlSafe, urlSafe3[i]));
assertTrue("standard decode uuid", Arrays.equals(decodedStandard, ids[i]));
assertTrue("url-safe1 decode uuid", Arrays.equals(decodedUrlSafe1, ids[i]));
assertTrue("url-safe2 decode uuid", Arrays.equals(decodedUrlSafe2, ids[i]));
assertTrue("url-safe3 decode uuid", Arrays.equals(decodedUrlSafe3, ids[i]));
}
}
public void testByteToStringVariations() throws DecoderException {
Base64 base64 = new Base64(0);
byte[] b1 = StringUtils.getBytesUtf8("Hello World");
byte[] b2 = new byte[0];
byte[] b3 = null;
byte[] b4 = Hex.decodeHex("2bf7cc2701fe4397b49ebeed5acc7090".toCharArray()); // for url-safe tests
assertEquals("byteToString Hello World", "SGVsbG8gV29ybGQ=", base64.encodeToString(b1));
assertEquals("byteToString static Hello World", "SGVsbG8gV29ybGQ=", Base64.encodeBase64String(b1));
assertEquals("byteToString \"\"", "", base64.encodeToString(b2));
assertEquals("byteToString static \"\"", "", Base64.encodeBase64String(b2));
assertEquals("byteToString null", null, base64.encodeToString(b3));
assertEquals("byteToString static null", null, Base64.encodeBase64String(b3));
assertEquals("byteToString UUID", "K/fMJwH+Q5e0nr7tWsxwkA==", base64.encodeToString(b4));
assertEquals("byteToString static UUID", "K/fMJwH+Q5e0nr7tWsxwkA==", Base64.encodeBase64String(b4));
assertEquals("byteToString static-url-safe UUID", "K_fMJwH-Q5e0nr7tWsxwkA", Base64.encodeBase64URLSafeString(b4));
}
public void testStringToByteVariations() throws DecoderException {
Base64 base64 = new Base64();
String s1 = "SGVsbG8gV29ybGQ=\r\n";
String s2 = "";
String s3 = null;
String s4a = "K/fMJwH+Q5e0nr7tWsxwkA==\r\n";
String s4b = "K_fMJwH-Q5e0nr7tWsxwkA";
byte[] b4 = Hex.decodeHex("2bf7cc2701fe4397b49ebeed5acc7090".toCharArray()); // for url-safe tests
assertEquals("StringToByte Hello World", "Hello World", StringUtils.newStringUtf8(base64.decode(s1)));
assertEquals("StringToByte Hello World", "Hello World", StringUtils.newStringUtf8((byte[])base64.decode((Object)s1)));
assertEquals("StringToByte static Hello World", "Hello World", StringUtils.newStringUtf8(Base64.decodeBase64(s1)));
assertEquals("StringToByte \"\"", "", StringUtils.newStringUtf8(base64.decode(s2)));
assertEquals("StringToByte static \"\"", "", StringUtils.newStringUtf8(Base64.decodeBase64(s2)));
assertEquals("StringToByte null", null, StringUtils.newStringUtf8(base64.decode(s3)));
assertEquals("StringToByte static null", null, StringUtils.newStringUtf8(Base64.decodeBase64(s3)));
assertTrue("StringToByte UUID", Arrays.equals(b4, base64.decode(s4b)));
assertTrue("StringToByte static UUID", Arrays.equals(b4, Base64.decodeBase64(s4a)));
assertTrue("StringToByte static-url-safe UUID", Arrays.equals(b4, Base64.decodeBase64(s4b)));
}
private String toString(byte[] data) {
StringBuffer buf = new StringBuffer();
for (int i = 0; i < data.length; i++) {
buf.append(data[i]);
if (i != data.length - 1) {
buf.append(",");
}
}
return buf.toString();
}
} | // You are a professional Java test case writer, please create a test case named `testCodec112` for the issue `Codec-CODEC-112`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Codec-CODEC-112
//
// ## Issue-Title:
// Base64.encodeBase64(byte[] binaryData, boolean isChunked, boolean urlSafe, int maxResultSize) throws IAE for valid maxResultSize if isChunked is false
//
// ## Issue-Description:
//
// If isChunked is false, Base64.encodeBase64(byte[] binaryData, boolean isChunked, boolean urlSafe, int maxResultSize) throws IAE for valid maxResultSize.
//
//
// Test case and fix will be applied shortly.
//
//
//
//
//
public void testCodec112() {
| 343 | 9 | 339 | src/test/org/apache/commons/codec/binary/Base64Test.java | src/test | ```markdown
## Issue-ID: Codec-CODEC-112
## Issue-Title:
Base64.encodeBase64(byte[] binaryData, boolean isChunked, boolean urlSafe, int maxResultSize) throws IAE for valid maxResultSize if isChunked is false
## Issue-Description:
If isChunked is false, Base64.encodeBase64(byte[] binaryData, boolean isChunked, boolean urlSafe, int maxResultSize) throws IAE for valid maxResultSize.
Test case and fix will be applied shortly.
```
You are a professional Java test case writer, please create a test case named `testCodec112` for the issue `Codec-CODEC-112`, utilizing the provided issue report information and the following function signature.
```java
public void testCodec112() {
```
| 339 | [
"org.apache.commons.codec.binary.Base64"
] | 7d90f257853357655db3f4c468a96d64ff6f3d7ebf6f5fb9794c7a692a1d8ed3 | public void testCodec112() | // You are a professional Java test case writer, please create a test case named `testCodec112` for the issue `Codec-CODEC-112`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Codec-CODEC-112
//
// ## Issue-Title:
// Base64.encodeBase64(byte[] binaryData, boolean isChunked, boolean urlSafe, int maxResultSize) throws IAE for valid maxResultSize if isChunked is false
//
// ## Issue-Description:
//
// If isChunked is false, Base64.encodeBase64(byte[] binaryData, boolean isChunked, boolean urlSafe, int maxResultSize) throws IAE for valid maxResultSize.
//
//
// Test case and fix will be applied shortly.
//
//
//
//
//
| Codec | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.codec.binary;
import java.io.UnsupportedEncodingException;
import java.math.BigInteger;
import java.util.Arrays;
import java.util.Random;
import junit.framework.TestCase;
import org.apache.commons.codec.DecoderException;
import org.apache.commons.codec.EncoderException;
/**
* Test cases for Base64 class.
*
* @see <a href="http://www.ietf.org/rfc/rfc2045.txt">RFC 2045</a>
* @author Apache Software Foundation
* @version $Id$
*/
public class Base64Test extends TestCase {
private Random _random = new Random();
/**
* Construct a new instance of this test case.
*
* @param name
* Name of the test case
*/
public Base64Test(String name) {
super(name);
}
/**
* @return Returns the _random.
*/
public Random getRandom() {
return this._random;
}
/**
* Test the isStringBase64 method.
*/
public void testIsStringBase64() {
String nullString = null;
String emptyString = "";
String validString = "abc===defg\n\r123456\r789\r\rABC\n\nDEF==GHI\r\nJKL==============";
String invalidString = validString + ((char)0); // append null character
try {
Base64.isBase64(nullString);
fail("Base64.isStringBase64() should not be null-safe.");
} catch (NullPointerException npe) {
assertNotNull("Base64.isStringBase64() should not be null-safe.", npe);
}
assertTrue("Base64.isStringBase64(empty-string) is true", Base64.isBase64(emptyString));
assertTrue("Base64.isStringBase64(valid-string) is true", Base64.isBase64(validString));
assertFalse("Base64.isStringBase64(invalid-string) is false", Base64.isBase64(invalidString));
}
/**
* Test the Base64 implementation
*/
public void testBase64() {
String content = "Hello World";
String encodedContent;
byte[] encodedBytes = Base64.encodeBase64(StringUtils.getBytesUtf8(content));
encodedContent = StringUtils.newStringUtf8(encodedBytes);
assertTrue("encoding hello world", encodedContent.equals("SGVsbG8gV29ybGQ="));
Base64 b64 = new Base64(Base64.MIME_CHUNK_SIZE, null); // null lineSeparator same as saying no-chunking
encodedBytes = b64.encode(StringUtils.getBytesUtf8(content));
encodedContent = StringUtils.newStringUtf8(encodedBytes);
assertTrue("encoding hello world", encodedContent.equals("SGVsbG8gV29ybGQ="));
b64 = new Base64(0, null); // null lineSeparator same as saying no-chunking
encodedBytes = b64.encode(StringUtils.getBytesUtf8(content));
encodedContent = StringUtils.newStringUtf8(encodedBytes);
assertTrue("encoding hello world", encodedContent.equals("SGVsbG8gV29ybGQ="));
// bogus characters to decode (to skip actually)
byte[] decode = b64.decode("SGVsbG{������}8gV29ybGQ=");
String decodeString = StringUtils.newStringUtf8(decode);
assertTrue("decode hello world", decodeString.equals("Hello World"));
}
/**
* Tests Base64.encodeBase64().
*/
public void testChunkedEncodeMultipleOf76() {
byte[] expectedEncode = Base64.encodeBase64(Base64TestData.DECODED, true);
// convert to "\r\n" so we're equal to the old openssl encoding test stored
// in Base64TestData.ENCODED_76_CHARS_PER_LINE:
String actualResult = Base64TestData.ENCODED_76_CHARS_PER_LINE.replaceAll("\n", "\r\n");
byte[] actualEncode = StringUtils.getBytesUtf8(actualResult);
assertTrue("chunkedEncodeMultipleOf76", Arrays.equals(expectedEncode, actualEncode));
}
/**
* CODEC-68: isBase64 throws ArrayIndexOutOfBoundsException on some non-BASE64 bytes
*/
public void testCodec68() {
byte[] x = new byte[]{'n', 'A', '=', '=', (byte) 0x9c};
Base64.decodeBase64(x);
}
public void testCodeInteger1() throws UnsupportedEncodingException {
String encodedInt1 = "li7dzDacuo67Jg7mtqEm2TRuOMU=";
BigInteger bigInt1 = new BigInteger("85739377120809420210425962799" + "0318636601332086981");
assertEquals(encodedInt1, new String(Base64.encodeInteger(bigInt1)));
assertEquals(bigInt1, Base64.decodeInteger(encodedInt1.getBytes("UTF-8")));
}
public void testCodeInteger2() throws UnsupportedEncodingException {
String encodedInt2 = "9B5ypLY9pMOmtxCeTDHgwdNFeGs=";
BigInteger bigInt2 = new BigInteger("13936727572861167254666467268" + "91466679477132949611");
assertEquals(encodedInt2, new String(Base64.encodeInteger(bigInt2)));
assertEquals(bigInt2, Base64.decodeInteger(encodedInt2.getBytes("UTF-8")));
}
public void testCodeInteger3() throws UnsupportedEncodingException {
String encodedInt3 = "FKIhdgaG5LGKiEtF1vHy4f3y700zaD6QwDS3IrNVGzNp2" + "rY+1LFWTK6D44AyiC1n8uWz1itkYMZF0/aKDK0Yjg==";
BigInteger bigInt3 = new BigInteger("10806548154093873461951748545"
+ "1196989136416448805819079363524309897749044958112417136240557"
+ "4495062430572478766856090958495998158114332651671116876320938126");
assertEquals(encodedInt3, new String(Base64.encodeInteger(bigInt3)));
assertEquals(bigInt3, Base64.decodeInteger(encodedInt3.getBytes("UTF-8")));
}
public void testCodeInteger4() throws UnsupportedEncodingException {
String encodedInt4 = "ctA8YGxrtngg/zKVvqEOefnwmViFztcnPBYPlJsvh6yKI"
+ "4iDm68fnp4Mi3RrJ6bZAygFrUIQLxLjV+OJtgJAEto0xAs+Mehuq1DkSFEpP3o"
+ "DzCTOsrOiS1DwQe4oIb7zVk/9l7aPtJMHW0LVlMdwZNFNNJoqMcT2ZfCPrfvYv"
+ "Q0=";
BigInteger bigInt4 = new BigInteger("80624726256040348115552042320"
+ "6968135001872753709424419772586693950232350200555646471175944"
+ "519297087885987040810778908507262272892702303774422853675597"
+ "748008534040890923814202286633163248086055216976551456088015"
+ "338880713818192088877057717530169381044092839402438015097654"
+ "53542091716518238707344493641683483917");
assertEquals(encodedInt4, new String(Base64.encodeInteger(bigInt4)));
assertEquals(bigInt4, Base64.decodeInteger(encodedInt4.getBytes("UTF-8")));
}
public void testCodeIntegerEdgeCases() {
// TODO
}
public void testCodeIntegerNull() {
try {
Base64.encodeInteger(null);
fail("Exception not thrown when passing in null to encodeInteger(BigInteger)");
} catch (NullPointerException npe) {
// expected
} catch (Exception e) {
fail("Incorrect Exception caught when passing in null to encodeInteger(BigInteger)");
}
}
public void testConstructors() {
Base64 base64;
base64 = new Base64();
base64 = new Base64(-1);
base64 = new Base64(-1, new byte[]{});
base64 = new Base64(64, new byte[]{});
try {
base64 = new Base64(-1, new byte[]{'A'});
fail("Should have rejected attempt to use 'A' as a line separator");
} catch (IllegalArgumentException ignored) {
// Expected
}
try {
base64 = new Base64(64, new byte[]{'A'});
fail("Should have rejected attempt to use 'A' as a line separator");
} catch (IllegalArgumentException ignored) {
// Expected
}
try {
base64 = new Base64(64, new byte[]{'='});
fail("Should have rejected attempt to use '=' as a line separator");
} catch (IllegalArgumentException ignored) {
// Expected
}
base64 = new Base64(64, new byte[]{'$'}); // OK
try {
base64 = new Base64(64, new byte[]{'A', '$'});
fail("Should have rejected attempt to use 'A$' as a line separator");
} catch (IllegalArgumentException ignored) {
// Expected
}
base64 = new Base64(64, new byte[]{' ', '$', '\n', '\r', '\t'}); // OK
assertNotNull(base64);
}
public void testConstructor_Int_ByteArray_Boolean() {
Base64 base64 = new Base64(65, new byte[]{'\t'}, false);
byte[] encoded = base64.encode(Base64TestData.DECODED);
String expectedResult = Base64TestData.ENCODED_64_CHARS_PER_LINE;
expectedResult = expectedResult.replace('\n', '\t');
String result = StringUtils.newStringUtf8(encoded);
assertEquals("new Base64(65, \\t, false)", expectedResult, result);
}
public void testConstructor_Int_ByteArray_Boolean_UrlSafe() {
// url-safe variation
Base64 base64 = new Base64(64, new byte[]{'\t'}, true);
byte[] encoded = base64.encode(Base64TestData.DECODED);
String expectedResult = Base64TestData.ENCODED_64_CHARS_PER_LINE;
expectedResult = expectedResult.replaceAll("=", ""); // url-safe has no == padding.
expectedResult = expectedResult.replace('\n', '\t');
expectedResult = expectedResult.replace('+', '-');
expectedResult = expectedResult.replace('/', '_');
String result = StringUtils.newStringUtf8(encoded);
assertEquals("new Base64(64, \\t, true)", result, expectedResult);
}
/**
* Tests conditional true branch for "marker0" test.
*/
public void testDecodePadMarkerIndex2() throws UnsupportedEncodingException {
assertEquals("A", new String(Base64.decodeBase64("QQ==".getBytes("UTF-8"))));
}
/**
* Tests conditional branches for "marker1" test.
*/
public void testDecodePadMarkerIndex3() throws UnsupportedEncodingException {
assertEquals("AA", new String(Base64.decodeBase64("QUE=".getBytes("UTF-8"))));
assertEquals("AAA", new String(Base64.decodeBase64("QUFB".getBytes("UTF-8"))));
}
public void testDecodePadOnly() throws UnsupportedEncodingException {
assertTrue(Base64.decodeBase64("====".getBytes("UTF-8")).length == 0);
assertEquals("", new String(Base64.decodeBase64("====".getBytes("UTF-8"))));
// Test truncated padding
assertTrue(Base64.decodeBase64("===".getBytes("UTF-8")).length == 0);
assertTrue(Base64.decodeBase64("==".getBytes("UTF-8")).length == 0);
assertTrue(Base64.decodeBase64("=".getBytes("UTF-8")).length == 0);
assertTrue(Base64.decodeBase64("".getBytes("UTF-8")).length == 0);
}
public void testDecodePadOnlyChunked() throws UnsupportedEncodingException {
assertTrue(Base64.decodeBase64("====\n".getBytes("UTF-8")).length == 0);
assertEquals("", new String(Base64.decodeBase64("====\n".getBytes("UTF-8"))));
// Test truncated padding
assertTrue(Base64.decodeBase64("===\n".getBytes("UTF-8")).length == 0);
assertTrue(Base64.decodeBase64("==\n".getBytes("UTF-8")).length == 0);
assertTrue(Base64.decodeBase64("=\n".getBytes("UTF-8")).length == 0);
assertTrue(Base64.decodeBase64("\n".getBytes("UTF-8")).length == 0);
}
public void testDecodeWithWhitespace() throws Exception {
String orig = "I am a late night coder.";
byte[] encodedArray = Base64.encodeBase64(orig.getBytes("UTF-8"));
StringBuffer intermediate = new StringBuffer(new String(encodedArray));
intermediate.insert(2, ' ');
intermediate.insert(5, '\t');
intermediate.insert(10, '\r');
intermediate.insert(15, '\n');
byte[] encodedWithWS = intermediate.toString().getBytes("UTF-8");
byte[] decodedWithWS = Base64.decodeBase64(encodedWithWS);
String dest = new String(decodedWithWS);
assertTrue("Dest string doesn't equal the original", dest.equals(orig));
}
/**
* Test encode and decode of empty byte array.
*/
public void testEmptyBase64() {
byte[] empty = new byte[0];
byte[] result = Base64.encodeBase64(empty);
assertEquals("empty base64 encode", 0, result.length);
assertEquals("empty base64 encode", null, Base64.encodeBase64(null));
empty = new byte[0];
result = Base64.decodeBase64(empty);
assertEquals("empty base64 decode", 0, result.length);
assertEquals("empty base64 encode", null, Base64.decodeBase64((byte[]) null));
}
// encode/decode a large random array
public void testEncodeDecodeRandom() {
for (int i = 1; i < 5; i++) {
byte[] data = new byte[this.getRandom().nextInt(10000) + 1];
this.getRandom().nextBytes(data);
byte[] enc = Base64.encodeBase64(data);
assertTrue(Base64.isBase64(enc));
byte[] data2 = Base64.decodeBase64(enc);
assertTrue(Arrays.equals(data, data2));
}
}
// encode/decode random arrays from size 0 to size 11
public void testEncodeDecodeSmall() {
for (int i = 0; i < 12; i++) {
byte[] data = new byte[i];
this.getRandom().nextBytes(data);
byte[] enc = Base64.encodeBase64(data);
assertTrue("\"" + (new String(enc)) + "\" is Base64 data.", Base64.isBase64(enc));
byte[] data2 = Base64.decodeBase64(enc);
assertTrue(toString(data) + " equals " + toString(data2), Arrays.equals(data, data2));
}
}
public void testEncodeOverMaxSize() throws Exception {
testEncodeOverMaxSize(-1);
testEncodeOverMaxSize(0);
testEncodeOverMaxSize(1);
testEncodeOverMaxSize(2);
}
public void testCodec112() { // size calculation assumes always chunked
byte[] in = new byte[] {0};
byte[] out=Base64.encodeBase64(in);
Base64.encodeBase64(in, false, false, out.length);
}
private void testEncodeOverMaxSize(int maxSize) throws Exception {
try {
Base64.encodeBase64(Base64TestData.DECODED, true, false, maxSize);
fail("Expected " + IllegalArgumentException.class.getName());
} catch (IllegalArgumentException e) {
// Expected
}
}
public void testIgnoringNonBase64InDecode() throws Exception {
assertEquals("The quick brown fox jumped over the lazy dogs.", new String(Base64
.decodeBase64("VGhlIH@$#$@%F1aWN@#@#@@rIGJyb3duIGZve\n\r\t%#%#%#%CBqd##$#$W1wZWQgb3ZlciB0aGUgbGF6eSBkb2dzLg==".getBytes("UTF-8"))));
}
public void testIsArrayByteBase64() {
assertFalse(Base64.isBase64(new byte[]{Byte.MIN_VALUE}));
assertFalse(Base64.isBase64(new byte[]{-125}));
assertFalse(Base64.isBase64(new byte[]{-10}));
assertFalse(Base64.isBase64(new byte[]{0}));
assertFalse(Base64.isBase64(new byte[]{64, Byte.MAX_VALUE}));
assertFalse(Base64.isBase64(new byte[]{Byte.MAX_VALUE}));
assertTrue(Base64.isBase64(new byte[]{'A'}));
assertFalse(Base64.isBase64(new byte[]{'A', Byte.MIN_VALUE}));
assertTrue(Base64.isBase64(new byte[]{'A', 'Z', 'a'}));
assertTrue(Base64.isBase64(new byte[]{'/', '=', '+'}));
assertFalse(Base64.isBase64(new byte[]{'$'}));
}
/**
* Tests isUrlSafe.
*/
public void testIsUrlSafe() {
Base64 base64Standard = new Base64(false);
Base64 base64URLSafe = new Base64(true);
assertFalse("Base64.isUrlSafe=false", base64Standard.isUrlSafe());
assertTrue("Base64.isUrlSafe=true", base64URLSafe.isUrlSafe());
byte[] whiteSpace = {' ', '\n', '\r', '\t'};
assertTrue("Base64.isBase64(whiteSpace)=true", Base64.isBase64(whiteSpace));
}
public void testKnownDecodings() throws UnsupportedEncodingException {
assertEquals("The quick brown fox jumped over the lazy dogs.", new String(Base64
.decodeBase64("VGhlIHF1aWNrIGJyb3duIGZveCBqdW1wZWQgb3ZlciB0aGUgbGF6eSBkb2dzLg==".getBytes("UTF-8"))));
assertEquals("It was the best of times, it was the worst of times.", new String(Base64
.decodeBase64("SXQgd2FzIHRoZSBiZXN0IG9mIHRpbWVzLCBpdCB3YXMgdGhlIHdvcnN0IG9mIHRpbWVzLg==".getBytes("UTF-8"))));
assertEquals("http://jakarta.apache.org/commmons", new String(Base64
.decodeBase64("aHR0cDovL2pha2FydGEuYXBhY2hlLm9yZy9jb21tbW9ucw==".getBytes("UTF-8"))));
assertEquals("AaBbCcDdEeFfGgHhIiJjKkLlMmNnOoPpQqRrSsTtUuVvWwXxYyZz", new String(Base64
.decodeBase64("QWFCYkNjRGRFZUZmR2dIaElpSmpLa0xsTW1Obk9vUHBRcVJyU3NUdFV1VnZXd1h4WXlaeg==".getBytes("UTF-8"))));
assertEquals("{ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9 }", new String(Base64.decodeBase64("eyAwLCAxLCAyLCAzLCA0LCA1LCA2LCA3LCA4LCA5IH0="
.getBytes("UTF-8"))));
assertEquals("xyzzy!", new String(Base64.decodeBase64("eHl6enkh".getBytes("UTF-8"))));
}
public void testKnownEncodings() throws UnsupportedEncodingException {
assertEquals("VGhlIHF1aWNrIGJyb3duIGZveCBqdW1wZWQgb3ZlciB0aGUgbGF6eSBkb2dzLg==", new String(Base64
.encodeBase64("The quick brown fox jumped over the lazy dogs.".getBytes("UTF-8"))));
assertEquals(
"YmxhaCBibGFoIGJsYWggYmxhaCBibGFoIGJsYWggYmxhaCBibGFoIGJsYWggYmxhaCBibGFoIGJs\r\nYWggYmxhaCBibGFoIGJsYWggYmxhaCBibGFoIGJsYWggYmxhaCBibGFoIGJsYWggYmxhaCBibGFo\r\nIGJsYWggYmxhaCBibGFoIGJsYWggYmxhaCBibGFoIGJsYWggYmxhaCBibGFoIGJsYWggYmxhaCBi\r\nbGFoIGJsYWg=\r\n",
new String(
Base64
.encodeBase64Chunked("blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah blah"
.getBytes("UTF-8"))));
assertEquals("SXQgd2FzIHRoZSBiZXN0IG9mIHRpbWVzLCBpdCB3YXMgdGhlIHdvcnN0IG9mIHRpbWVzLg==", new String(Base64
.encodeBase64("It was the best of times, it was the worst of times.".getBytes("UTF-8"))));
assertEquals("aHR0cDovL2pha2FydGEuYXBhY2hlLm9yZy9jb21tbW9ucw==", new String(Base64
.encodeBase64("http://jakarta.apache.org/commmons".getBytes("UTF-8"))));
assertEquals("QWFCYkNjRGRFZUZmR2dIaElpSmpLa0xsTW1Obk9vUHBRcVJyU3NUdFV1VnZXd1h4WXlaeg==", new String(Base64
.encodeBase64("AaBbCcDdEeFfGgHhIiJjKkLlMmNnOoPpQqRrSsTtUuVvWwXxYyZz".getBytes("UTF-8"))));
assertEquals("eyAwLCAxLCAyLCAzLCA0LCA1LCA2LCA3LCA4LCA5IH0=", new String(Base64.encodeBase64("{ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9 }"
.getBytes("UTF-8"))));
assertEquals("eHl6enkh", new String(Base64.encodeBase64("xyzzy!".getBytes("UTF-8"))));
}
public void testNonBase64Test() throws Exception {
byte[] bArray = {'%'};
assertFalse("Invalid Base64 array was incorrectly validated as " + "an array of Base64 encoded data", Base64
.isBase64(bArray));
try {
Base64 b64 = new Base64();
byte[] result = b64.decode(bArray);
assertTrue("The result should be empty as the test encoded content did " + "not contain any valid base 64 characters",
result.length == 0);
} catch (Exception e) {
fail("Exception was thrown when trying to decode "
+ "invalid base64 encoded data - RFC 2045 requires that all "
+ "non base64 character be discarded, an exception should not"
+ " have been thrown");
}
}
public void testObjectDecodeWithInvalidParameter() throws Exception {
Base64 b64 = new Base64();
try {
b64.decode(new Integer(5));
fail("decode(Object) didn't throw an exception when passed an Integer object");
} catch (DecoderException e) {
// ignored
}
}
public void testObjectDecodeWithValidParameter() throws Exception {
String original = "Hello World!";
Object o = Base64.encodeBase64(original.getBytes("UTF-8"));
Base64 b64 = new Base64();
Object oDecoded = b64.decode(o);
byte[] baDecoded = (byte[]) oDecoded;
String dest = new String(baDecoded);
assertTrue("dest string does not equal original", dest.equals(original));
}
public void testObjectEncodeWithInvalidParameter() throws Exception {
Base64 b64 = new Base64();
try {
b64.encode("Yadayadayada");
fail("encode(Object) didn't throw an exception when passed a String object");
} catch (EncoderException e) {
// Expected
}
}
public void testObjectEncodeWithValidParameter() throws Exception {
String original = "Hello World!";
Object origObj = original.getBytes("UTF-8");
Base64 b64 = new Base64();
Object oEncoded = b64.encode(origObj);
byte[] bArray = Base64.decodeBase64((byte[]) oEncoded);
String dest = new String(bArray);
assertTrue("dest string does not equal original", dest.equals(original));
}
public void testObjectEncode() throws Exception {
Base64 b64 = new Base64();
assertEquals("SGVsbG8gV29ybGQ=", new String(b64.encode("Hello World".getBytes("UTF-8"))));
}
public void testPairs() {
assertEquals("AAA=", new String(Base64.encodeBase64(new byte[]{0, 0})));
for (int i = -128; i <= 127; i++) {
byte test[] = {(byte) i, (byte) i};
assertTrue(Arrays.equals(test, Base64.decodeBase64(Base64.encodeBase64(test))));
}
}
/**
* Tests RFC 2045 section 2.1 CRLF definition.
*/
public void testRfc2045Section2Dot1CrLfDefinition() {
assertTrue(Arrays.equals(new byte[]{13, 10}, Base64.CHUNK_SEPARATOR));
}
/**
* Tests RFC 2045 section 6.8 chuck size definition.
*/
public void testRfc2045Section6Dot8ChunkSizeDefinition() {
assertEquals(76, Base64.MIME_CHUNK_SIZE);
}
/**
* Tests RFC 1421 section 4.3.2.4 chuck size definition.
*/
public void testRfc1421Section6Dot8ChunkSizeDefinition() {
assertEquals(64, Base64.PEM_CHUNK_SIZE);
}
/**
* Tests RFC 4648 section 10 test vectors.
* <ul>
* <li>BASE64("") = ""</li>
* <li>BASE64("f") = "Zg=="</li>
* <li>BASE64("fo") = "Zm8="</li>
* <li>BASE64("foo") = "Zm9v"</li>
* <li>BASE64("foob") = "Zm9vYg=="</li>
* <li>BASE64("fooba") = "Zm9vYmE="</li>
* <li>BASE64("foobar") = "Zm9vYmFy"</li>
* </ul>
*
* @see <a href="http://tools.ietf.org/html/rfc4648">http://tools.ietf.org/html/rfc4648</a>
*/
public void testRfc4648Section10Decode() {
assertEquals("", StringUtils.newStringUsAscii(Base64.decodeBase64("")));
assertEquals("f", StringUtils.newStringUsAscii(Base64.decodeBase64("Zg==")));
assertEquals("fo", StringUtils.newStringUsAscii(Base64.decodeBase64("Zm8=")));
assertEquals("foo", StringUtils.newStringUsAscii(Base64.decodeBase64("Zm9v")));
assertEquals("foob", StringUtils.newStringUsAscii(Base64.decodeBase64("Zm9vYg==")));
assertEquals("fooba", StringUtils.newStringUsAscii(Base64.decodeBase64("Zm9vYmE=")));
assertEquals("foobar", StringUtils.newStringUsAscii(Base64.decodeBase64("Zm9vYmFy")));
}
/**
* Tests RFC 4648 section 10 test vectors.
* <ul>
* <li>BASE64("") = ""</li>
* <li>BASE64("f") = "Zg=="</li>
* <li>BASE64("fo") = "Zm8="</li>
* <li>BASE64("foo") = "Zm9v"</li>
* <li>BASE64("foob") = "Zm9vYg=="</li>
* <li>BASE64("fooba") = "Zm9vYmE="</li>
* <li>BASE64("foobar") = "Zm9vYmFy"</li>
* </ul>
*
* @see <a href="http://tools.ietf.org/html/rfc4648">http://tools.ietf.org/html/rfc4648</a>
*/
public void testRfc4648Section10DecodeWithCrLf() {
String CRLF = StringUtils.newStringUsAscii(Base64.CHUNK_SEPARATOR);
assertEquals("", StringUtils.newStringUsAscii(Base64.decodeBase64("" + CRLF)));
assertEquals("f", StringUtils.newStringUsAscii(Base64.decodeBase64("Zg==" + CRLF)));
assertEquals("fo", StringUtils.newStringUsAscii(Base64.decodeBase64("Zm8=" + CRLF)));
assertEquals("foo", StringUtils.newStringUsAscii(Base64.decodeBase64("Zm9v" + CRLF)));
assertEquals("foob", StringUtils.newStringUsAscii(Base64.decodeBase64("Zm9vYg==" + CRLF)));
assertEquals("fooba", StringUtils.newStringUsAscii(Base64.decodeBase64("Zm9vYmE=" + CRLF)));
assertEquals("foobar", StringUtils.newStringUsAscii(Base64.decodeBase64("Zm9vYmFy" + CRLF)));
}
/**
* Tests RFC 4648 section 10 test vectors.
* <ul>
* <li>BASE64("") = ""</li>
* <li>BASE64("f") = "Zg=="</li>
* <li>BASE64("fo") = "Zm8="</li>
* <li>BASE64("foo") = "Zm9v"</li>
* <li>BASE64("foob") = "Zm9vYg=="</li>
* <li>BASE64("fooba") = "Zm9vYmE="</li>
* <li>BASE64("foobar") = "Zm9vYmFy"</li>
* </ul>
*
* @see <a href="http://tools.ietf.org/html/rfc4648">http://tools.ietf.org/html/rfc4648</a>
*/
public void testRfc4648Section10Encode() {
assertEquals("", Base64.encodeBase64String(StringUtils.getBytesUtf8("")));
assertEquals("Zg==", Base64.encodeBase64String(StringUtils.getBytesUtf8("f")));
assertEquals("Zm8=", Base64.encodeBase64String(StringUtils.getBytesUtf8("fo")));
assertEquals("Zm9v", Base64.encodeBase64String(StringUtils.getBytesUtf8("foo")));
assertEquals("Zm9vYg==", Base64.encodeBase64String(StringUtils.getBytesUtf8("foob")));
assertEquals("Zm9vYmE=", Base64.encodeBase64String(StringUtils.getBytesUtf8("fooba")));
assertEquals("Zm9vYmFy", Base64.encodeBase64String(StringUtils.getBytesUtf8("foobar")));
}
/**
* Tests RFC 4648 section 10 test vectors.
* <ul>
* <li>BASE64("") = ""</li>
* <li>BASE64("f") = "Zg=="</li>
* <li>BASE64("fo") = "Zm8="</li>
* <li>BASE64("foo") = "Zm9v"</li>
* <li>BASE64("foob") = "Zm9vYg=="</li>
* <li>BASE64("fooba") = "Zm9vYmE="</li>
* <li>BASE64("foobar") = "Zm9vYmFy"</li>
* </ul>
*
* @see <a href="http://tools.ietf.org/html/rfc4648">http://tools.ietf.org/html/rfc4648</a>
*/
public void testRfc4648Section10DecodeEncode() {
testDecodeEncode("");
//testDecodeEncode("Zg==");
//testDecodeEncode("Zm8=");
//testDecodeEncode("Zm9v");
//testDecodeEncode("Zm9vYg==");
//testDecodeEncode("Zm9vYmE=");
//testDecodeEncode("Zm9vYmFy");
}
private void testDecodeEncode(String encodedText) {
String decodedText = StringUtils.newStringUsAscii(Base64.decodeBase64(encodedText));
String encodedText2 = Base64.encodeBase64String(StringUtils.getBytesUtf8(decodedText));
assertEquals(encodedText, encodedText2);
}
/**
* Tests RFC 4648 section 10 test vectors.
* <ul>
* <li>BASE64("") = ""</li>
* <li>BASE64("f") = "Zg=="</li>
* <li>BASE64("fo") = "Zm8="</li>
* <li>BASE64("foo") = "Zm9v"</li>
* <li>BASE64("foob") = "Zm9vYg=="</li>
* <li>BASE64("fooba") = "Zm9vYmE="</li>
* <li>BASE64("foobar") = "Zm9vYmFy"</li>
* </ul>
*
* @see <a href="http://tools.ietf.org/html/rfc4648">http://tools.ietf.org/html/rfc4648</a>
*/
public void testRfc4648Section10EncodeDecode() {
testEncodeDecode("");
testEncodeDecode("f");
testEncodeDecode("fo");
testEncodeDecode("foo");
testEncodeDecode("foob");
testEncodeDecode("fooba");
testEncodeDecode("foobar");
}
private void testEncodeDecode(String plainText) {
String encodedText = Base64.encodeBase64String(StringUtils.getBytesUtf8(plainText));
String decodedText = StringUtils.newStringUsAscii(Base64.decodeBase64(encodedText));
assertEquals(plainText, decodedText);
}
public void testSingletons() {
assertEquals("AA==", new String(Base64.encodeBase64(new byte[]{(byte) 0})));
assertEquals("AQ==", new String(Base64.encodeBase64(new byte[]{(byte) 1})));
assertEquals("Ag==", new String(Base64.encodeBase64(new byte[]{(byte) 2})));
assertEquals("Aw==", new String(Base64.encodeBase64(new byte[]{(byte) 3})));
assertEquals("BA==", new String(Base64.encodeBase64(new byte[]{(byte) 4})));
assertEquals("BQ==", new String(Base64.encodeBase64(new byte[]{(byte) 5})));
assertEquals("Bg==", new String(Base64.encodeBase64(new byte[]{(byte) 6})));
assertEquals("Bw==", new String(Base64.encodeBase64(new byte[]{(byte) 7})));
assertEquals("CA==", new String(Base64.encodeBase64(new byte[]{(byte) 8})));
assertEquals("CQ==", new String(Base64.encodeBase64(new byte[]{(byte) 9})));
assertEquals("Cg==", new String(Base64.encodeBase64(new byte[]{(byte) 10})));
assertEquals("Cw==", new String(Base64.encodeBase64(new byte[]{(byte) 11})));
assertEquals("DA==", new String(Base64.encodeBase64(new byte[]{(byte) 12})));
assertEquals("DQ==", new String(Base64.encodeBase64(new byte[]{(byte) 13})));
assertEquals("Dg==", new String(Base64.encodeBase64(new byte[]{(byte) 14})));
assertEquals("Dw==", new String(Base64.encodeBase64(new byte[]{(byte) 15})));
assertEquals("EA==", new String(Base64.encodeBase64(new byte[]{(byte) 16})));
assertEquals("EQ==", new String(Base64.encodeBase64(new byte[]{(byte) 17})));
assertEquals("Eg==", new String(Base64.encodeBase64(new byte[]{(byte) 18})));
assertEquals("Ew==", new String(Base64.encodeBase64(new byte[]{(byte) 19})));
assertEquals("FA==", new String(Base64.encodeBase64(new byte[]{(byte) 20})));
assertEquals("FQ==", new String(Base64.encodeBase64(new byte[]{(byte) 21})));
assertEquals("Fg==", new String(Base64.encodeBase64(new byte[]{(byte) 22})));
assertEquals("Fw==", new String(Base64.encodeBase64(new byte[]{(byte) 23})));
assertEquals("GA==", new String(Base64.encodeBase64(new byte[]{(byte) 24})));
assertEquals("GQ==", new String(Base64.encodeBase64(new byte[]{(byte) 25})));
assertEquals("Gg==", new String(Base64.encodeBase64(new byte[]{(byte) 26})));
assertEquals("Gw==", new String(Base64.encodeBase64(new byte[]{(byte) 27})));
assertEquals("HA==", new String(Base64.encodeBase64(new byte[]{(byte) 28})));
assertEquals("HQ==", new String(Base64.encodeBase64(new byte[]{(byte) 29})));
assertEquals("Hg==", new String(Base64.encodeBase64(new byte[]{(byte) 30})));
assertEquals("Hw==", new String(Base64.encodeBase64(new byte[]{(byte) 31})));
assertEquals("IA==", new String(Base64.encodeBase64(new byte[]{(byte) 32})));
assertEquals("IQ==", new String(Base64.encodeBase64(new byte[]{(byte) 33})));
assertEquals("Ig==", new String(Base64.encodeBase64(new byte[]{(byte) 34})));
assertEquals("Iw==", new String(Base64.encodeBase64(new byte[]{(byte) 35})));
assertEquals("JA==", new String(Base64.encodeBase64(new byte[]{(byte) 36})));
assertEquals("JQ==", new String(Base64.encodeBase64(new byte[]{(byte) 37})));
assertEquals("Jg==", new String(Base64.encodeBase64(new byte[]{(byte) 38})));
assertEquals("Jw==", new String(Base64.encodeBase64(new byte[]{(byte) 39})));
assertEquals("KA==", new String(Base64.encodeBase64(new byte[]{(byte) 40})));
assertEquals("KQ==", new String(Base64.encodeBase64(new byte[]{(byte) 41})));
assertEquals("Kg==", new String(Base64.encodeBase64(new byte[]{(byte) 42})));
assertEquals("Kw==", new String(Base64.encodeBase64(new byte[]{(byte) 43})));
assertEquals("LA==", new String(Base64.encodeBase64(new byte[]{(byte) 44})));
assertEquals("LQ==", new String(Base64.encodeBase64(new byte[]{(byte) 45})));
assertEquals("Lg==", new String(Base64.encodeBase64(new byte[]{(byte) 46})));
assertEquals("Lw==", new String(Base64.encodeBase64(new byte[]{(byte) 47})));
assertEquals("MA==", new String(Base64.encodeBase64(new byte[]{(byte) 48})));
assertEquals("MQ==", new String(Base64.encodeBase64(new byte[]{(byte) 49})));
assertEquals("Mg==", new String(Base64.encodeBase64(new byte[]{(byte) 50})));
assertEquals("Mw==", new String(Base64.encodeBase64(new byte[]{(byte) 51})));
assertEquals("NA==", new String(Base64.encodeBase64(new byte[]{(byte) 52})));
assertEquals("NQ==", new String(Base64.encodeBase64(new byte[]{(byte) 53})));
assertEquals("Ng==", new String(Base64.encodeBase64(new byte[]{(byte) 54})));
assertEquals("Nw==", new String(Base64.encodeBase64(new byte[]{(byte) 55})));
assertEquals("OA==", new String(Base64.encodeBase64(new byte[]{(byte) 56})));
assertEquals("OQ==", new String(Base64.encodeBase64(new byte[]{(byte) 57})));
assertEquals("Og==", new String(Base64.encodeBase64(new byte[]{(byte) 58})));
assertEquals("Ow==", new String(Base64.encodeBase64(new byte[]{(byte) 59})));
assertEquals("PA==", new String(Base64.encodeBase64(new byte[]{(byte) 60})));
assertEquals("PQ==", new String(Base64.encodeBase64(new byte[]{(byte) 61})));
assertEquals("Pg==", new String(Base64.encodeBase64(new byte[]{(byte) 62})));
assertEquals("Pw==", new String(Base64.encodeBase64(new byte[]{(byte) 63})));
assertEquals("QA==", new String(Base64.encodeBase64(new byte[]{(byte) 64})));
assertEquals("QQ==", new String(Base64.encodeBase64(new byte[]{(byte) 65})));
assertEquals("Qg==", new String(Base64.encodeBase64(new byte[]{(byte) 66})));
assertEquals("Qw==", new String(Base64.encodeBase64(new byte[]{(byte) 67})));
assertEquals("RA==", new String(Base64.encodeBase64(new byte[]{(byte) 68})));
assertEquals("RQ==", new String(Base64.encodeBase64(new byte[]{(byte) 69})));
assertEquals("Rg==", new String(Base64.encodeBase64(new byte[]{(byte) 70})));
assertEquals("Rw==", new String(Base64.encodeBase64(new byte[]{(byte) 71})));
assertEquals("SA==", new String(Base64.encodeBase64(new byte[]{(byte) 72})));
assertEquals("SQ==", new String(Base64.encodeBase64(new byte[]{(byte) 73})));
assertEquals("Sg==", new String(Base64.encodeBase64(new byte[]{(byte) 74})));
assertEquals("Sw==", new String(Base64.encodeBase64(new byte[]{(byte) 75})));
assertEquals("TA==", new String(Base64.encodeBase64(new byte[]{(byte) 76})));
assertEquals("TQ==", new String(Base64.encodeBase64(new byte[]{(byte) 77})));
assertEquals("Tg==", new String(Base64.encodeBase64(new byte[]{(byte) 78})));
assertEquals("Tw==", new String(Base64.encodeBase64(new byte[]{(byte) 79})));
assertEquals("UA==", new String(Base64.encodeBase64(new byte[]{(byte) 80})));
assertEquals("UQ==", new String(Base64.encodeBase64(new byte[]{(byte) 81})));
assertEquals("Ug==", new String(Base64.encodeBase64(new byte[]{(byte) 82})));
assertEquals("Uw==", new String(Base64.encodeBase64(new byte[]{(byte) 83})));
assertEquals("VA==", new String(Base64.encodeBase64(new byte[]{(byte) 84})));
assertEquals("VQ==", new String(Base64.encodeBase64(new byte[]{(byte) 85})));
assertEquals("Vg==", new String(Base64.encodeBase64(new byte[]{(byte) 86})));
assertEquals("Vw==", new String(Base64.encodeBase64(new byte[]{(byte) 87})));
assertEquals("WA==", new String(Base64.encodeBase64(new byte[]{(byte) 88})));
assertEquals("WQ==", new String(Base64.encodeBase64(new byte[]{(byte) 89})));
assertEquals("Wg==", new String(Base64.encodeBase64(new byte[]{(byte) 90})));
assertEquals("Ww==", new String(Base64.encodeBase64(new byte[]{(byte) 91})));
assertEquals("XA==", new String(Base64.encodeBase64(new byte[]{(byte) 92})));
assertEquals("XQ==", new String(Base64.encodeBase64(new byte[]{(byte) 93})));
assertEquals("Xg==", new String(Base64.encodeBase64(new byte[]{(byte) 94})));
assertEquals("Xw==", new String(Base64.encodeBase64(new byte[]{(byte) 95})));
assertEquals("YA==", new String(Base64.encodeBase64(new byte[]{(byte) 96})));
assertEquals("YQ==", new String(Base64.encodeBase64(new byte[]{(byte) 97})));
assertEquals("Yg==", new String(Base64.encodeBase64(new byte[]{(byte) 98})));
assertEquals("Yw==", new String(Base64.encodeBase64(new byte[]{(byte) 99})));
assertEquals("ZA==", new String(Base64.encodeBase64(new byte[]{(byte) 100})));
assertEquals("ZQ==", new String(Base64.encodeBase64(new byte[]{(byte) 101})));
assertEquals("Zg==", new String(Base64.encodeBase64(new byte[]{(byte) 102})));
assertEquals("Zw==", new String(Base64.encodeBase64(new byte[]{(byte) 103})));
assertEquals("aA==", new String(Base64.encodeBase64(new byte[]{(byte) 104})));
for (int i = -128; i <= 127; i++) {
byte test[] = {(byte) i};
assertTrue(Arrays.equals(test, Base64.decodeBase64(Base64.encodeBase64(test))));
}
}
public void testSingletonsChunked() {
assertEquals("AA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0})));
assertEquals("AQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 1})));
assertEquals("Ag==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 2})));
assertEquals("Aw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 3})));
assertEquals("BA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 4})));
assertEquals("BQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 5})));
assertEquals("Bg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 6})));
assertEquals("Bw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 7})));
assertEquals("CA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 8})));
assertEquals("CQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 9})));
assertEquals("Cg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 10})));
assertEquals("Cw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 11})));
assertEquals("DA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 12})));
assertEquals("DQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 13})));
assertEquals("Dg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 14})));
assertEquals("Dw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 15})));
assertEquals("EA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 16})));
assertEquals("EQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 17})));
assertEquals("Eg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 18})));
assertEquals("Ew==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 19})));
assertEquals("FA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 20})));
assertEquals("FQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 21})));
assertEquals("Fg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 22})));
assertEquals("Fw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 23})));
assertEquals("GA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 24})));
assertEquals("GQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 25})));
assertEquals("Gg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 26})));
assertEquals("Gw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 27})));
assertEquals("HA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 28})));
assertEquals("HQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 29})));
assertEquals("Hg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 30})));
assertEquals("Hw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 31})));
assertEquals("IA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 32})));
assertEquals("IQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 33})));
assertEquals("Ig==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 34})));
assertEquals("Iw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 35})));
assertEquals("JA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 36})));
assertEquals("JQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 37})));
assertEquals("Jg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 38})));
assertEquals("Jw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 39})));
assertEquals("KA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 40})));
assertEquals("KQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 41})));
assertEquals("Kg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 42})));
assertEquals("Kw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 43})));
assertEquals("LA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 44})));
assertEquals("LQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 45})));
assertEquals("Lg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 46})));
assertEquals("Lw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 47})));
assertEquals("MA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 48})));
assertEquals("MQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 49})));
assertEquals("Mg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 50})));
assertEquals("Mw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 51})));
assertEquals("NA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 52})));
assertEquals("NQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 53})));
assertEquals("Ng==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 54})));
assertEquals("Nw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 55})));
assertEquals("OA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 56})));
assertEquals("OQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 57})));
assertEquals("Og==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 58})));
assertEquals("Ow==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 59})));
assertEquals("PA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 60})));
assertEquals("PQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 61})));
assertEquals("Pg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 62})));
assertEquals("Pw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 63})));
assertEquals("QA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 64})));
assertEquals("QQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 65})));
assertEquals("Qg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 66})));
assertEquals("Qw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 67})));
assertEquals("RA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 68})));
assertEquals("RQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 69})));
assertEquals("Rg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 70})));
assertEquals("Rw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 71})));
assertEquals("SA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 72})));
assertEquals("SQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 73})));
assertEquals("Sg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 74})));
assertEquals("Sw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 75})));
assertEquals("TA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 76})));
assertEquals("TQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 77})));
assertEquals("Tg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 78})));
assertEquals("Tw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 79})));
assertEquals("UA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 80})));
assertEquals("UQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 81})));
assertEquals("Ug==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 82})));
assertEquals("Uw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 83})));
assertEquals("VA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 84})));
assertEquals("VQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 85})));
assertEquals("Vg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 86})));
assertEquals("Vw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 87})));
assertEquals("WA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 88})));
assertEquals("WQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 89})));
assertEquals("Wg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 90})));
assertEquals("Ww==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 91})));
assertEquals("XA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 92})));
assertEquals("XQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 93})));
assertEquals("Xg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 94})));
assertEquals("Xw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 95})));
assertEquals("YA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 96})));
assertEquals("YQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 97})));
assertEquals("Yg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 98})));
assertEquals("Yw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 99})));
assertEquals("ZA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 100})));
assertEquals("ZQ==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 101})));
assertEquals("Zg==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 102})));
assertEquals("Zw==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 103})));
assertEquals("aA==\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 104})));
}
public void testTriplets() {
assertEquals("AAAA", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 0})));
assertEquals("AAAB", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 1})));
assertEquals("AAAC", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 2})));
assertEquals("AAAD", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 3})));
assertEquals("AAAE", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 4})));
assertEquals("AAAF", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 5})));
assertEquals("AAAG", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 6})));
assertEquals("AAAH", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 7})));
assertEquals("AAAI", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 8})));
assertEquals("AAAJ", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 9})));
assertEquals("AAAK", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 10})));
assertEquals("AAAL", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 11})));
assertEquals("AAAM", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 12})));
assertEquals("AAAN", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 13})));
assertEquals("AAAO", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 14})));
assertEquals("AAAP", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 15})));
assertEquals("AAAQ", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 16})));
assertEquals("AAAR", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 17})));
assertEquals("AAAS", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 18})));
assertEquals("AAAT", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 19})));
assertEquals("AAAU", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 20})));
assertEquals("AAAV", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 21})));
assertEquals("AAAW", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 22})));
assertEquals("AAAX", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 23})));
assertEquals("AAAY", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 24})));
assertEquals("AAAZ", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 25})));
assertEquals("AAAa", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 26})));
assertEquals("AAAb", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 27})));
assertEquals("AAAc", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 28})));
assertEquals("AAAd", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 29})));
assertEquals("AAAe", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 30})));
assertEquals("AAAf", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 31})));
assertEquals("AAAg", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 32})));
assertEquals("AAAh", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 33})));
assertEquals("AAAi", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 34})));
assertEquals("AAAj", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 35})));
assertEquals("AAAk", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 36})));
assertEquals("AAAl", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 37})));
assertEquals("AAAm", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 38})));
assertEquals("AAAn", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 39})));
assertEquals("AAAo", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 40})));
assertEquals("AAAp", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 41})));
assertEquals("AAAq", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 42})));
assertEquals("AAAr", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 43})));
assertEquals("AAAs", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 44})));
assertEquals("AAAt", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 45})));
assertEquals("AAAu", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 46})));
assertEquals("AAAv", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 47})));
assertEquals("AAAw", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 48})));
assertEquals("AAAx", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 49})));
assertEquals("AAAy", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 50})));
assertEquals("AAAz", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 51})));
assertEquals("AAA0", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 52})));
assertEquals("AAA1", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 53})));
assertEquals("AAA2", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 54})));
assertEquals("AAA3", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 55})));
assertEquals("AAA4", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 56})));
assertEquals("AAA5", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 57})));
assertEquals("AAA6", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 58})));
assertEquals("AAA7", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 59})));
assertEquals("AAA8", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 60})));
assertEquals("AAA9", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 61})));
assertEquals("AAA+", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 62})));
assertEquals("AAA/", new String(Base64.encodeBase64(new byte[]{(byte) 0, (byte) 0, (byte) 63})));
}
public void testTripletsChunked() {
assertEquals("AAAA\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 0})));
assertEquals("AAAB\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 1})));
assertEquals("AAAC\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 2})));
assertEquals("AAAD\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 3})));
assertEquals("AAAE\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 4})));
assertEquals("AAAF\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 5})));
assertEquals("AAAG\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 6})));
assertEquals("AAAH\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 7})));
assertEquals("AAAI\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 8})));
assertEquals("AAAJ\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 9})));
assertEquals("AAAK\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 10})));
assertEquals("AAAL\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 11})));
assertEquals("AAAM\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 12})));
assertEquals("AAAN\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 13})));
assertEquals("AAAO\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 14})));
assertEquals("AAAP\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 15})));
assertEquals("AAAQ\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 16})));
assertEquals("AAAR\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 17})));
assertEquals("AAAS\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 18})));
assertEquals("AAAT\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 19})));
assertEquals("AAAU\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 20})));
assertEquals("AAAV\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 21})));
assertEquals("AAAW\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 22})));
assertEquals("AAAX\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 23})));
assertEquals("AAAY\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 24})));
assertEquals("AAAZ\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 25})));
assertEquals("AAAa\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 26})));
assertEquals("AAAb\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 27})));
assertEquals("AAAc\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 28})));
assertEquals("AAAd\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 29})));
assertEquals("AAAe\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 30})));
assertEquals("AAAf\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 31})));
assertEquals("AAAg\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 32})));
assertEquals("AAAh\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 33})));
assertEquals("AAAi\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 34})));
assertEquals("AAAj\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 35})));
assertEquals("AAAk\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 36})));
assertEquals("AAAl\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 37})));
assertEquals("AAAm\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 38})));
assertEquals("AAAn\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 39})));
assertEquals("AAAo\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 40})));
assertEquals("AAAp\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 41})));
assertEquals("AAAq\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 42})));
assertEquals("AAAr\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 43})));
assertEquals("AAAs\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 44})));
assertEquals("AAAt\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 45})));
assertEquals("AAAu\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 46})));
assertEquals("AAAv\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 47})));
assertEquals("AAAw\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 48})));
assertEquals("AAAx\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 49})));
assertEquals("AAAy\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 50})));
assertEquals("AAAz\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 51})));
assertEquals("AAA0\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 52})));
assertEquals("AAA1\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 53})));
assertEquals("AAA2\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 54})));
assertEquals("AAA3\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 55})));
assertEquals("AAA4\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 56})));
assertEquals("AAA5\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 57})));
assertEquals("AAA6\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 58})));
assertEquals("AAA7\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 59})));
assertEquals("AAA8\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 60})));
assertEquals("AAA9\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 61})));
assertEquals("AAA+\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 62})));
assertEquals("AAA/\r\n", new String(Base64.encodeBase64Chunked(new byte[]{(byte) 0, (byte) 0, (byte) 63})));
}
/**
* Tests url-safe Base64 against random data, sizes 0 to 150.
*/
public void testUrlSafe() {
// test random data of sizes 0 thru 150
for (int i = 0; i <= 150; i++) {
byte[][] randomData = Base64TestData.randomData(i, true);
byte[] encoded = randomData[1];
byte[] decoded = randomData[0];
byte[] result = Base64.decodeBase64(encoded);
assertTrue("url-safe i=" + i, Arrays.equals(decoded, result));
assertFalse("url-safe i=" + i + " no '='", Base64TestData.bytesContain(encoded, (byte) '='));
assertFalse("url-safe i=" + i + " no '\\'", Base64TestData.bytesContain(encoded, (byte) '\\'));
assertFalse("url-safe i=" + i + " no '+'", Base64TestData.bytesContain(encoded, (byte) '+'));
}
}
/**
* Base64 encoding of UUID's is a common use-case, especially in URL-SAFE mode. This test case ends up being the
* "URL-SAFE" JUnit's.
*
* @throws DecoderException
* if Hex.decode() fails - a serious problem since Hex comes from our own commons-codec!
*/
public void testUUID() throws DecoderException {
// The 4 UUID's below contains mixtures of + and / to help us test the
// URL-SAFE encoding mode.
byte[][] ids = new byte[4][];
// ids[0] was chosen so that it encodes with at least one +.
ids[0] = Hex.decodeHex("94ed8d0319e4493399560fb67404d370".toCharArray());
// ids[1] was chosen so that it encodes with both / and +.
ids[1] = Hex.decodeHex("2bf7cc2701fe4397b49ebeed5acc7090".toCharArray());
// ids[2] was chosen so that it encodes with at least one /.
ids[2] = Hex.decodeHex("64be154b6ffa40258d1a01288e7c31ca".toCharArray());
// ids[3] was chosen so that it encodes with both / and +, with /
// right at the beginning.
ids[3] = Hex.decodeHex("ff7f8fc01cdb471a8c8b5a9306183fe8".toCharArray());
byte[][] standard = new byte[4][];
standard[0] = StringUtils.getBytesUtf8("lO2NAxnkSTOZVg+2dATTcA==");
standard[1] = StringUtils.getBytesUtf8("K/fMJwH+Q5e0nr7tWsxwkA==");
standard[2] = StringUtils.getBytesUtf8("ZL4VS2/6QCWNGgEojnwxyg==");
standard[3] = StringUtils.getBytesUtf8("/3+PwBzbRxqMi1qTBhg/6A==");
byte[][] urlSafe1 = new byte[4][];
// regular padding (two '==' signs).
urlSafe1[0] = StringUtils.getBytesUtf8("lO2NAxnkSTOZVg-2dATTcA==");
urlSafe1[1] = StringUtils.getBytesUtf8("K_fMJwH-Q5e0nr7tWsxwkA==");
urlSafe1[2] = StringUtils.getBytesUtf8("ZL4VS2_6QCWNGgEojnwxyg==");
urlSafe1[3] = StringUtils.getBytesUtf8("_3-PwBzbRxqMi1qTBhg_6A==");
byte[][] urlSafe2 = new byte[4][];
// single padding (only one '=' sign).
urlSafe2[0] = StringUtils.getBytesUtf8("lO2NAxnkSTOZVg-2dATTcA=");
urlSafe2[1] = StringUtils.getBytesUtf8("K_fMJwH-Q5e0nr7tWsxwkA=");
urlSafe2[2] = StringUtils.getBytesUtf8("ZL4VS2_6QCWNGgEojnwxyg=");
urlSafe2[3] = StringUtils.getBytesUtf8("_3-PwBzbRxqMi1qTBhg_6A=");
byte[][] urlSafe3 = new byte[4][];
// no padding (no '=' signs).
urlSafe3[0] = StringUtils.getBytesUtf8("lO2NAxnkSTOZVg-2dATTcA");
urlSafe3[1] = StringUtils.getBytesUtf8("K_fMJwH-Q5e0nr7tWsxwkA");
urlSafe3[2] = StringUtils.getBytesUtf8("ZL4VS2_6QCWNGgEojnwxyg");
urlSafe3[3] = StringUtils.getBytesUtf8("_3-PwBzbRxqMi1qTBhg_6A");
for (int i = 0; i < 4; i++) {
byte[] encodedStandard = Base64.encodeBase64(ids[i]);
byte[] encodedUrlSafe = Base64.encodeBase64URLSafe(ids[i]);
byte[] decodedStandard = Base64.decodeBase64(standard[i]);
byte[] decodedUrlSafe1 = Base64.decodeBase64(urlSafe1[i]);
byte[] decodedUrlSafe2 = Base64.decodeBase64(urlSafe2[i]);
byte[] decodedUrlSafe3 = Base64.decodeBase64(urlSafe3[i]);
// Very important debugging output should anyone
// ever need to delve closely into this stuff.
if (false) {
System.out.println("reference: [" + Hex.encodeHexString(ids[i]) + "]");
System.out.println("standard: [" +
Hex.encodeHexString(decodedStandard) +
"] From: [" +
StringUtils.newStringUtf8(standard[i]) +
"]");
System.out.println("safe1: [" +
Hex.encodeHexString(decodedUrlSafe1) +
"] From: [" +
StringUtils.newStringUtf8(urlSafe1[i]) +
"]");
System.out.println("safe2: [" +
Hex.encodeHexString(decodedUrlSafe2) +
"] From: [" +
StringUtils.newStringUtf8(urlSafe2[i]) +
"]");
System.out.println("safe3: [" +
Hex.encodeHexString(decodedUrlSafe3) +
"] From: [" +
StringUtils.newStringUtf8(urlSafe3[i]) +
"]");
}
assertTrue("standard encode uuid", Arrays.equals(encodedStandard, standard[i]));
assertTrue("url-safe encode uuid", Arrays.equals(encodedUrlSafe, urlSafe3[i]));
assertTrue("standard decode uuid", Arrays.equals(decodedStandard, ids[i]));
assertTrue("url-safe1 decode uuid", Arrays.equals(decodedUrlSafe1, ids[i]));
assertTrue("url-safe2 decode uuid", Arrays.equals(decodedUrlSafe2, ids[i]));
assertTrue("url-safe3 decode uuid", Arrays.equals(decodedUrlSafe3, ids[i]));
}
}
public void testByteToStringVariations() throws DecoderException {
Base64 base64 = new Base64(0);
byte[] b1 = StringUtils.getBytesUtf8("Hello World");
byte[] b2 = new byte[0];
byte[] b3 = null;
byte[] b4 = Hex.decodeHex("2bf7cc2701fe4397b49ebeed5acc7090".toCharArray()); // for url-safe tests
assertEquals("byteToString Hello World", "SGVsbG8gV29ybGQ=", base64.encodeToString(b1));
assertEquals("byteToString static Hello World", "SGVsbG8gV29ybGQ=", Base64.encodeBase64String(b1));
assertEquals("byteToString \"\"", "", base64.encodeToString(b2));
assertEquals("byteToString static \"\"", "", Base64.encodeBase64String(b2));
assertEquals("byteToString null", null, base64.encodeToString(b3));
assertEquals("byteToString static null", null, Base64.encodeBase64String(b3));
assertEquals("byteToString UUID", "K/fMJwH+Q5e0nr7tWsxwkA==", base64.encodeToString(b4));
assertEquals("byteToString static UUID", "K/fMJwH+Q5e0nr7tWsxwkA==", Base64.encodeBase64String(b4));
assertEquals("byteToString static-url-safe UUID", "K_fMJwH-Q5e0nr7tWsxwkA", Base64.encodeBase64URLSafeString(b4));
}
public void testStringToByteVariations() throws DecoderException {
Base64 base64 = new Base64();
String s1 = "SGVsbG8gV29ybGQ=\r\n";
String s2 = "";
String s3 = null;
String s4a = "K/fMJwH+Q5e0nr7tWsxwkA==\r\n";
String s4b = "K_fMJwH-Q5e0nr7tWsxwkA";
byte[] b4 = Hex.decodeHex("2bf7cc2701fe4397b49ebeed5acc7090".toCharArray()); // for url-safe tests
assertEquals("StringToByte Hello World", "Hello World", StringUtils.newStringUtf8(base64.decode(s1)));
assertEquals("StringToByte Hello World", "Hello World", StringUtils.newStringUtf8((byte[])base64.decode((Object)s1)));
assertEquals("StringToByte static Hello World", "Hello World", StringUtils.newStringUtf8(Base64.decodeBase64(s1)));
assertEquals("StringToByte \"\"", "", StringUtils.newStringUtf8(base64.decode(s2)));
assertEquals("StringToByte static \"\"", "", StringUtils.newStringUtf8(Base64.decodeBase64(s2)));
assertEquals("StringToByte null", null, StringUtils.newStringUtf8(base64.decode(s3)));
assertEquals("StringToByte static null", null, StringUtils.newStringUtf8(Base64.decodeBase64(s3)));
assertTrue("StringToByte UUID", Arrays.equals(b4, base64.decode(s4b)));
assertTrue("StringToByte static UUID", Arrays.equals(b4, Base64.decodeBase64(s4a)));
assertTrue("StringToByte static-url-safe UUID", Arrays.equals(b4, Base64.decodeBase64(s4b)));
}
private String toString(byte[] data) {
StringBuffer buf = new StringBuffer();
for (int i = 0; i < data.length; i++) {
buf.append(data[i]);
if (i != data.length - 1) {
buf.append(",");
}
}
return buf.toString();
}
} |
||
public void testDontRemoveBreakInTryFinally() throws Exception {
foldSame("function f() {b:try{throw 9} finally {break b} return 1;}");
} | com.google.javascript.jscomp.MinimizeExitPointsTest::testDontRemoveBreakInTryFinally | test/com/google/javascript/jscomp/MinimizeExitPointsTest.java | 276 | test/com/google/javascript/jscomp/MinimizeExitPointsTest.java | testDontRemoveBreakInTryFinally | /*
* Copyright 2009 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import com.google.javascript.rhino.Node;
/**
* @author [email protected] (John Lenz)
*/
public class MinimizeExitPointsTest extends CompilerTestCase {
@Override
public void setUp() {
super.enableLineNumberCheck(true);
}
@Override
protected CompilerPass getProcessor(final Compiler compiler) {
return new CompilerPass() {
@Override
public void process(Node externs, Node js) {
NodeTraversal.traverse(compiler, js, new MinimizeExitPoints(compiler));
}
};
}
@Override
protected int getNumRepetitions() {
return 1;
}
void foldSame(String js) {
testSame(js);
}
void fold(String js, String expected) {
test(js, expected);
}
void fold(String js, String expected, DiagnosticType warning) {
test(js, expected, warning);
}
public void testBreakOptimization() throws Exception {
fold("f:{if(true){a();break f;}else;b();}",
"f:{if(true){a()}else{b()}}");
fold("f:{if(false){a();break f;}else;b();break f;}",
"f:{if(false){a()}else{b()}}");
fold("f:{if(a()){b();break f;}else;c();}",
"f:{if(a()){b();}else{c();}}");
fold("f:{if(a()){b()}else{c();break f;}}",
"f:{if(a()){b()}else{c();}}");
fold("f:{if(a()){b();break f;}else;}",
"f:{if(a()){b();}else;}");
fold("f:{if(a()){break f;}else;}",
"f:{if(a()){}else;}");
fold("f:while(a())break f;",
"f:while(a())break f");
foldSame("f:for(x in a())break f");
fold("f:{while(a())break;}",
"f:{while(a())break;}");
foldSame("f:{for(x in a())break}");
fold("f:try{break f;}catch(e){break f;}",
"f:try{}catch(e){}");
fold("f:try{if(a()){break f;}else{break f;} break f;}catch(e){}",
"f:try{if(a()){}else{}}catch(e){}");
fold("f:g:break f",
"");
fold("f:g:{if(a()){break f;}else{break f;} break f;}",
"f:g:{if(a()){}else{}}");
}
public void testFunctionReturnOptimization() throws Exception {
fold("function f(){if(a()){b();if(c())return;}}",
"function f(){if(a()){b();if(c());}}");
fold("function f(){if(x)return; x=3; return; }",
"function f(){if(x); else x=3}");
fold("function f(){if(true){a();return;}else;b();}",
"function f(){if(true){a();}else{b();}}");
fold("function f(){if(false){a();return;}else;b();return;}",
"function f(){if(false){a();}else{b();}}");
fold("function f(){if(a()){b();return;}else;c();}",
"function f(){if(a()){b();}else{c();}}");
fold("function f(){if(a()){b()}else{c();return;}}",
"function f(){if(a()){b()}else{c();}}");
fold("function f(){if(a()){b();return;}else;}",
"function f(){if(a()){b();}else;}");
fold("function f(){if(a()){return;}else{return;} return;}",
"function f(){if(a()){}else{}}");
fold("function f(){if(a()){return;}else{return;} b();}",
"function f(){if(a()){}else{return;b()}}");
fold("function f(){ if (x) return; if (y) return; if (z) return; w(); }",
" function f() {" +
" if (x) {} else { if (y) {} else { if (z) {} else w(); }}" +
" }");
fold("function f(){while(a())return;}",
"function f(){while(a())return}");
foldSame("function f(){for(x in a())return}");
fold("function f(){while(a())break;}",
"function f(){while(a())break}");
foldSame("function f(){for(x in a())break}");
fold("function f(){try{return;}catch(e){throw 9;}finally{return}}",
"function f(){try{}catch(e){throw 9;}finally{return}}");
foldSame("function f(){try{throw 9;}finally{return;}}");
fold("function f(){try{return;}catch(e){return;}}",
"function f(){try{}catch(e){}}");
fold("function f(){try{if(a()){return;}else{return;} return;}catch(e){}}",
"function f(){try{if(a()){}else{}}catch(e){}}");
fold("function f(){g:return}",
"function f(){}");
fold("function f(){g:if(a()){return;}else{return;} return;}",
"function f(){g:if(a()){}else{}}");
fold("function f(){try{g:if(a()){throw 9;} return;}finally{return}}",
"function f(){try{g:if(a()){throw 9;}}finally{return}}");
}
public void testWhileContinueOptimization() throws Exception {
fold("while(true){if(x)continue; x=3; continue; }",
"while(true)if(x);else x=3");
foldSame("while(true){a();continue;b();}");
fold("while(true){if(true){a();continue;}else;b();}",
"while(true){if(true){a();}else{b()}}");
fold("while(true){if(false){a();continue;}else;b();continue;}",
"while(true){if(false){a()}else{b();}}");
fold("while(true){if(a()){b();continue;}else;c();}",
"while(true){if(a()){b();}else{c();}}");
fold("while(true){if(a()){b();}else{c();continue;}}",
"while(true){if(a()){b();}else{c();}}");
fold("while(true){if(a()){b();continue;}else;}",
"while(true){if(a()){b();}else;}");
fold("while(true){if(a()){continue;}else{continue;} continue;}",
"while(true){if(a()){}else{}}");
fold("while(true){if(a()){continue;}else{continue;} b();}",
"while(true){if(a()){}else{continue;b();}}");
fold("while(true)while(a())continue;",
"while(true)while(a());");
fold("while(true)for(x in a())continue",
"while(true)for(x in a());");
fold("while(true)while(a())break;",
"while(true)while(a())break");
fold("while(true)for(x in a())break",
"while(true)for(x in a())break");
fold("while(true){try{continue;}catch(e){continue;}}",
"while(true){try{}catch(e){}}");
fold("while(true){try{if(a()){continue;}else{continue;}" +
"continue;}catch(e){}}",
"while(true){try{if(a()){}else{}}catch(e){}}");
fold("while(true){g:continue}",
"while(true){}");
// This case could be improved.
fold("while(true){g:if(a()){continue;}else{continue;} continue;}",
"while(true){g:if(a());else;}");
}
public void testDoContinueOptimization() throws Exception {
fold("do{if(x)continue; x=3; continue; }while(true)",
"do if(x); else x=3; while(true)");
foldSame("do{a();continue;b()}while(true)");
fold("do{if(true){a();continue;}else;b();}while(true)",
"do{if(true){a();}else{b();}}while(true)");
fold("do{if(false){a();continue;}else;b();continue;}while(true)",
"do{if(false){a();}else{b();}}while(true)");
fold("do{if(a()){b();continue;}else;c();}while(true)",
"do{if(a()){b();}else{c()}}while(true)");
fold("do{if(a()){b();}else{c();continue;}}while(true)",
"do{if(a()){b();}else{c();}}while(true)");
fold("do{if(a()){b();continue;}else;}while(true)",
"do{if(a()){b();}else;}while(true)");
fold("do{if(a()){continue;}else{continue;} continue;}while(true)",
"do{if(a()){}else{}}while(true)");
fold("do{if(a()){continue;}else{continue;} b();}while(true)",
"do{if(a()){}else{continue; b();}}while(true)");
fold("do{while(a())continue;}while(true)",
"do while(a());while(true)");
fold("do{for(x in a())continue}while(true)",
"do for(x in a());while(true)");
fold("do{while(a())break;}while(true)",
"do while(a())break;while(true)");
fold("do for(x in a())break;while(true)",
"do for(x in a())break;while(true)");
fold("do{try{continue;}catch(e){continue;}}while(true)",
"do{try{}catch(e){}}while(true)");
fold("do{try{if(a()){continue;}else{continue;}" +
"continue;}catch(e){}}while(true)",
"do{try{if(a()){}else{}}catch(e){}}while(true)");
fold("do{g:continue}while(true)",
"do{}while(true)");
// This case could be improved.
fold("do{g:if(a()){continue;}else{continue;} continue;}while(true)",
"do{g:if(a());else;}while(true)");
fold("do { foo(); continue; } while(false)",
"do { foo(); } while(false)");
fold("do { foo(); break; } while(false)",
"do { foo(); } while(false)");
}
public void testForContinueOptimization() throws Exception {
fold("for(x in y){if(x)continue; x=3; continue; }",
"for(x in y)if(x);else x=3");
foldSame("for(x in y){a();continue;b()}");
fold("for(x in y){if(true){a();continue;}else;b();}",
"for(x in y){if(true)a();else b();}");
fold("for(x in y){if(false){a();continue;}else;b();continue;}",
"for(x in y){if(false){a();}else{b()}}");
fold("for(x in y){if(a()){b();continue;}else;c();}",
"for(x in y){if(a()){b();}else{c();}}");
fold("for(x in y){if(a()){b();}else{c();continue;}}",
"for(x in y){if(a()){b();}else{c();}}");
fold("for(x=0;x<y;x++){if(a()){b();continue;}else;}",
"for(x=0;x<y;x++){if(a()){b();}else;}");
fold("for(x=0;x<y;x++){if(a()){continue;}else{continue;} continue;}",
"for(x=0;x<y;x++){if(a()){}else{}}");
fold("for(x=0;x<y;x++){if(a()){continue;}else{continue;} b();}",
"for(x=0;x<y;x++){if(a()){}else{continue; b();}}");
fold("for(x=0;x<y;x++)while(a())continue;",
"for(x=0;x<y;x++)while(a());");
fold("for(x=0;x<y;x++)for(x in a())continue",
"for(x=0;x<y;x++)for(x in a());");
fold("for(x=0;x<y;x++)while(a())break;",
"for(x=0;x<y;x++)while(a())break");
foldSame("for(x=0;x<y;x++)for(x in a())break");
fold("for(x=0;x<y;x++){try{continue;}catch(e){continue;}}",
"for(x=0;x<y;x++){try{}catch(e){}}");
fold("for(x=0;x<y;x++){try{if(a()){continue;}else{continue;}" +
"continue;}catch(e){}}",
"for(x=0;x<y;x++){try{if(a()){}else{}}catch(e){}}");
fold("for(x=0;x<y;x++){g:continue}",
"for(x=0;x<y;x++){}");
// This case could be improved.
fold("for(x=0;x<y;x++){g:if(a()){continue;}else{continue;} continue;}",
"for(x=0;x<y;x++){g:if(a());else;}");
}
public void testCodeMotionDoesntBreakFunctionHoisting() throws Exception {
fold("function f() { if (x) return; foo(); function foo() {} }",
"function f() { if (x); else { function foo() {} foo(); } }");
}
public void testDontRemoveBreakInTryFinally() throws Exception {
foldSame("function f() {b:try{throw 9} finally {break b} return 1;}");
}
} | // You are a professional Java test case writer, please create a test case named `testDontRemoveBreakInTryFinally` for the issue `Closure-936`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-936
//
// ## Issue-Title:
// Break in finally block isn't optimized properly
//
// ## Issue-Description:
// b: try { throw("throw me") } finally { /\* fake catcher \*/ ; break b }; console.log("ok then...")
//
// ... gets optimized into ...
//
// throw"throw me";
//
// ... which is not the same.
//
// The break in the finally block should prevent the exception from being passed on. The expected result is:
//
// console.log("ok then...")
//
// ECMA-262 says:
//
// The production TryStatement : try Block Finally is evaluated as follows:
//
// Let B be the result of evaluating Block.
// Let F be the result of evaluating Finally.
// If F.type is normal, return B.
// Return F.
//
// F.type in this case would be 'break' and not 'normal', so 'break' overrides the 'throw' of B
//
// This is with the build available for download on Feb 28 2013.
//
//
public void testDontRemoveBreakInTryFinally() throws Exception {
| 276 | 126 | 274 | test/com/google/javascript/jscomp/MinimizeExitPointsTest.java | test | ```markdown
## Issue-ID: Closure-936
## Issue-Title:
Break in finally block isn't optimized properly
## Issue-Description:
b: try { throw("throw me") } finally { /\* fake catcher \*/ ; break b }; console.log("ok then...")
... gets optimized into ...
throw"throw me";
... which is not the same.
The break in the finally block should prevent the exception from being passed on. The expected result is:
console.log("ok then...")
ECMA-262 says:
The production TryStatement : try Block Finally is evaluated as follows:
Let B be the result of evaluating Block.
Let F be the result of evaluating Finally.
If F.type is normal, return B.
Return F.
F.type in this case would be 'break' and not 'normal', so 'break' overrides the 'throw' of B
This is with the build available for download on Feb 28 2013.
```
You are a professional Java test case writer, please create a test case named `testDontRemoveBreakInTryFinally` for the issue `Closure-936`, utilizing the provided issue report information and the following function signature.
```java
public void testDontRemoveBreakInTryFinally() throws Exception {
```
| 274 | [
"com.google.javascript.jscomp.MinimizeExitPoints"
] | 7d9d054f93de2de6f575788a8da1fb9a9d85ac5b8b629d4550c4174db46eaa84 | public void testDontRemoveBreakInTryFinally() throws Exception | // You are a professional Java test case writer, please create a test case named `testDontRemoveBreakInTryFinally` for the issue `Closure-936`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-936
//
// ## Issue-Title:
// Break in finally block isn't optimized properly
//
// ## Issue-Description:
// b: try { throw("throw me") } finally { /\* fake catcher \*/ ; break b }; console.log("ok then...")
//
// ... gets optimized into ...
//
// throw"throw me";
//
// ... which is not the same.
//
// The break in the finally block should prevent the exception from being passed on. The expected result is:
//
// console.log("ok then...")
//
// ECMA-262 says:
//
// The production TryStatement : try Block Finally is evaluated as follows:
//
// Let B be the result of evaluating Block.
// Let F be the result of evaluating Finally.
// If F.type is normal, return B.
// Return F.
//
// F.type in this case would be 'break' and not 'normal', so 'break' overrides the 'throw' of B
//
// This is with the build available for download on Feb 28 2013.
//
//
| Closure | /*
* Copyright 2009 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import com.google.javascript.rhino.Node;
/**
* @author [email protected] (John Lenz)
*/
public class MinimizeExitPointsTest extends CompilerTestCase {
@Override
public void setUp() {
super.enableLineNumberCheck(true);
}
@Override
protected CompilerPass getProcessor(final Compiler compiler) {
return new CompilerPass() {
@Override
public void process(Node externs, Node js) {
NodeTraversal.traverse(compiler, js, new MinimizeExitPoints(compiler));
}
};
}
@Override
protected int getNumRepetitions() {
return 1;
}
void foldSame(String js) {
testSame(js);
}
void fold(String js, String expected) {
test(js, expected);
}
void fold(String js, String expected, DiagnosticType warning) {
test(js, expected, warning);
}
public void testBreakOptimization() throws Exception {
fold("f:{if(true){a();break f;}else;b();}",
"f:{if(true){a()}else{b()}}");
fold("f:{if(false){a();break f;}else;b();break f;}",
"f:{if(false){a()}else{b()}}");
fold("f:{if(a()){b();break f;}else;c();}",
"f:{if(a()){b();}else{c();}}");
fold("f:{if(a()){b()}else{c();break f;}}",
"f:{if(a()){b()}else{c();}}");
fold("f:{if(a()){b();break f;}else;}",
"f:{if(a()){b();}else;}");
fold("f:{if(a()){break f;}else;}",
"f:{if(a()){}else;}");
fold("f:while(a())break f;",
"f:while(a())break f");
foldSame("f:for(x in a())break f");
fold("f:{while(a())break;}",
"f:{while(a())break;}");
foldSame("f:{for(x in a())break}");
fold("f:try{break f;}catch(e){break f;}",
"f:try{}catch(e){}");
fold("f:try{if(a()){break f;}else{break f;} break f;}catch(e){}",
"f:try{if(a()){}else{}}catch(e){}");
fold("f:g:break f",
"");
fold("f:g:{if(a()){break f;}else{break f;} break f;}",
"f:g:{if(a()){}else{}}");
}
public void testFunctionReturnOptimization() throws Exception {
fold("function f(){if(a()){b();if(c())return;}}",
"function f(){if(a()){b();if(c());}}");
fold("function f(){if(x)return; x=3; return; }",
"function f(){if(x); else x=3}");
fold("function f(){if(true){a();return;}else;b();}",
"function f(){if(true){a();}else{b();}}");
fold("function f(){if(false){a();return;}else;b();return;}",
"function f(){if(false){a();}else{b();}}");
fold("function f(){if(a()){b();return;}else;c();}",
"function f(){if(a()){b();}else{c();}}");
fold("function f(){if(a()){b()}else{c();return;}}",
"function f(){if(a()){b()}else{c();}}");
fold("function f(){if(a()){b();return;}else;}",
"function f(){if(a()){b();}else;}");
fold("function f(){if(a()){return;}else{return;} return;}",
"function f(){if(a()){}else{}}");
fold("function f(){if(a()){return;}else{return;} b();}",
"function f(){if(a()){}else{return;b()}}");
fold("function f(){ if (x) return; if (y) return; if (z) return; w(); }",
" function f() {" +
" if (x) {} else { if (y) {} else { if (z) {} else w(); }}" +
" }");
fold("function f(){while(a())return;}",
"function f(){while(a())return}");
foldSame("function f(){for(x in a())return}");
fold("function f(){while(a())break;}",
"function f(){while(a())break}");
foldSame("function f(){for(x in a())break}");
fold("function f(){try{return;}catch(e){throw 9;}finally{return}}",
"function f(){try{}catch(e){throw 9;}finally{return}}");
foldSame("function f(){try{throw 9;}finally{return;}}");
fold("function f(){try{return;}catch(e){return;}}",
"function f(){try{}catch(e){}}");
fold("function f(){try{if(a()){return;}else{return;} return;}catch(e){}}",
"function f(){try{if(a()){}else{}}catch(e){}}");
fold("function f(){g:return}",
"function f(){}");
fold("function f(){g:if(a()){return;}else{return;} return;}",
"function f(){g:if(a()){}else{}}");
fold("function f(){try{g:if(a()){throw 9;} return;}finally{return}}",
"function f(){try{g:if(a()){throw 9;}}finally{return}}");
}
public void testWhileContinueOptimization() throws Exception {
fold("while(true){if(x)continue; x=3; continue; }",
"while(true)if(x);else x=3");
foldSame("while(true){a();continue;b();}");
fold("while(true){if(true){a();continue;}else;b();}",
"while(true){if(true){a();}else{b()}}");
fold("while(true){if(false){a();continue;}else;b();continue;}",
"while(true){if(false){a()}else{b();}}");
fold("while(true){if(a()){b();continue;}else;c();}",
"while(true){if(a()){b();}else{c();}}");
fold("while(true){if(a()){b();}else{c();continue;}}",
"while(true){if(a()){b();}else{c();}}");
fold("while(true){if(a()){b();continue;}else;}",
"while(true){if(a()){b();}else;}");
fold("while(true){if(a()){continue;}else{continue;} continue;}",
"while(true){if(a()){}else{}}");
fold("while(true){if(a()){continue;}else{continue;} b();}",
"while(true){if(a()){}else{continue;b();}}");
fold("while(true)while(a())continue;",
"while(true)while(a());");
fold("while(true)for(x in a())continue",
"while(true)for(x in a());");
fold("while(true)while(a())break;",
"while(true)while(a())break");
fold("while(true)for(x in a())break",
"while(true)for(x in a())break");
fold("while(true){try{continue;}catch(e){continue;}}",
"while(true){try{}catch(e){}}");
fold("while(true){try{if(a()){continue;}else{continue;}" +
"continue;}catch(e){}}",
"while(true){try{if(a()){}else{}}catch(e){}}");
fold("while(true){g:continue}",
"while(true){}");
// This case could be improved.
fold("while(true){g:if(a()){continue;}else{continue;} continue;}",
"while(true){g:if(a());else;}");
}
public void testDoContinueOptimization() throws Exception {
fold("do{if(x)continue; x=3; continue; }while(true)",
"do if(x); else x=3; while(true)");
foldSame("do{a();continue;b()}while(true)");
fold("do{if(true){a();continue;}else;b();}while(true)",
"do{if(true){a();}else{b();}}while(true)");
fold("do{if(false){a();continue;}else;b();continue;}while(true)",
"do{if(false){a();}else{b();}}while(true)");
fold("do{if(a()){b();continue;}else;c();}while(true)",
"do{if(a()){b();}else{c()}}while(true)");
fold("do{if(a()){b();}else{c();continue;}}while(true)",
"do{if(a()){b();}else{c();}}while(true)");
fold("do{if(a()){b();continue;}else;}while(true)",
"do{if(a()){b();}else;}while(true)");
fold("do{if(a()){continue;}else{continue;} continue;}while(true)",
"do{if(a()){}else{}}while(true)");
fold("do{if(a()){continue;}else{continue;} b();}while(true)",
"do{if(a()){}else{continue; b();}}while(true)");
fold("do{while(a())continue;}while(true)",
"do while(a());while(true)");
fold("do{for(x in a())continue}while(true)",
"do for(x in a());while(true)");
fold("do{while(a())break;}while(true)",
"do while(a())break;while(true)");
fold("do for(x in a())break;while(true)",
"do for(x in a())break;while(true)");
fold("do{try{continue;}catch(e){continue;}}while(true)",
"do{try{}catch(e){}}while(true)");
fold("do{try{if(a()){continue;}else{continue;}" +
"continue;}catch(e){}}while(true)",
"do{try{if(a()){}else{}}catch(e){}}while(true)");
fold("do{g:continue}while(true)",
"do{}while(true)");
// This case could be improved.
fold("do{g:if(a()){continue;}else{continue;} continue;}while(true)",
"do{g:if(a());else;}while(true)");
fold("do { foo(); continue; } while(false)",
"do { foo(); } while(false)");
fold("do { foo(); break; } while(false)",
"do { foo(); } while(false)");
}
public void testForContinueOptimization() throws Exception {
fold("for(x in y){if(x)continue; x=3; continue; }",
"for(x in y)if(x);else x=3");
foldSame("for(x in y){a();continue;b()}");
fold("for(x in y){if(true){a();continue;}else;b();}",
"for(x in y){if(true)a();else b();}");
fold("for(x in y){if(false){a();continue;}else;b();continue;}",
"for(x in y){if(false){a();}else{b()}}");
fold("for(x in y){if(a()){b();continue;}else;c();}",
"for(x in y){if(a()){b();}else{c();}}");
fold("for(x in y){if(a()){b();}else{c();continue;}}",
"for(x in y){if(a()){b();}else{c();}}");
fold("for(x=0;x<y;x++){if(a()){b();continue;}else;}",
"for(x=0;x<y;x++){if(a()){b();}else;}");
fold("for(x=0;x<y;x++){if(a()){continue;}else{continue;} continue;}",
"for(x=0;x<y;x++){if(a()){}else{}}");
fold("for(x=0;x<y;x++){if(a()){continue;}else{continue;} b();}",
"for(x=0;x<y;x++){if(a()){}else{continue; b();}}");
fold("for(x=0;x<y;x++)while(a())continue;",
"for(x=0;x<y;x++)while(a());");
fold("for(x=0;x<y;x++)for(x in a())continue",
"for(x=0;x<y;x++)for(x in a());");
fold("for(x=0;x<y;x++)while(a())break;",
"for(x=0;x<y;x++)while(a())break");
foldSame("for(x=0;x<y;x++)for(x in a())break");
fold("for(x=0;x<y;x++){try{continue;}catch(e){continue;}}",
"for(x=0;x<y;x++){try{}catch(e){}}");
fold("for(x=0;x<y;x++){try{if(a()){continue;}else{continue;}" +
"continue;}catch(e){}}",
"for(x=0;x<y;x++){try{if(a()){}else{}}catch(e){}}");
fold("for(x=0;x<y;x++){g:continue}",
"for(x=0;x<y;x++){}");
// This case could be improved.
fold("for(x=0;x<y;x++){g:if(a()){continue;}else{continue;} continue;}",
"for(x=0;x<y;x++){g:if(a());else;}");
}
public void testCodeMotionDoesntBreakFunctionHoisting() throws Exception {
fold("function f() { if (x) return; foo(); function foo() {} }",
"function f() { if (x); else { function foo() {} foo(); } }");
}
public void testDontRemoveBreakInTryFinally() throws Exception {
foldSame("function f() {b:try{throw 9} finally {break b} return 1;}");
}
} |
||
@Test public void parsesQuiteRoughAttributes() {
String html = "<p =a>One<a =a";
Document doc = Jsoup.parse(html);
assertEquals("<p>One<a></a></p>", doc.body().html());
doc = Jsoup.parse("<p .....");
assertEquals("<p></p>", doc.body().html());
doc = Jsoup.parse("<p .....<p!!");
assertEquals("<p></p>\n<p></p>", doc.body().html());
} | org.jsoup.parser.ParserTest::parsesQuiteRoughAttributes | src/test/java/org/jsoup/parser/ParserTest.java | 52 | src/test/java/org/jsoup/parser/ParserTest.java | parsesQuiteRoughAttributes | package org.jsoup.parser;
import org.jsoup.Jsoup;
import org.jsoup.TextUtil;
import org.jsoup.nodes.Comment;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.nodes.TextNode;
import org.jsoup.select.Elements;
import org.junit.Test;
import java.util.List;
import static org.junit.Assert.*;
/**
Tests for the Parser
@author Jonathan Hedley, [email protected] */
public class ParserTest {
@Test public void parsesSimpleDocument() {
String html = "<html><head><title>First!</title></head><body><p>First post! <img src=\"foo.png\" /></p></body></html>";
Document doc = Jsoup.parse(html);
// need a better way to verify these:
Element p = doc.body().child(0);
assertEquals("p", p.tagName());
Element img = p.child(0);
assertEquals("foo.png", img.attr("src"));
assertEquals("img", img.tagName());
}
@Test public void parsesRoughAttributes() {
String html = "<html><head><title>First!</title></head><body><p class=\"foo > bar\">First post! <img src=\"foo.png\" /></p></body></html>";
Document doc = Jsoup.parse(html);
// need a better way to verify these:
Element p = doc.body().child(0);
assertEquals("p", p.tagName());
assertEquals("foo > bar", p.attr("class"));
}
@Test public void parsesQuiteRoughAttributes() {
String html = "<p =a>One<a =a";
Document doc = Jsoup.parse(html);
assertEquals("<p>One<a></a></p>", doc.body().html());
doc = Jsoup.parse("<p .....");
assertEquals("<p></p>", doc.body().html());
doc = Jsoup.parse("<p .....<p!!");
assertEquals("<p></p>\n<p></p>", doc.body().html());
}
@Test public void parsesComments() {
String html = "<html><head></head><body><img src=foo><!-- <table><tr><td></table> --><p>Hello</p></body></html>";
Document doc = Jsoup.parse(html);
Element body = doc.body();
Comment comment = (Comment) body.childNode(1); // comment should not be sub of img, as it's an empty tag
assertEquals(" <table><tr><td></table> ", comment.getData());
Element p = body.child(1);
TextNode text = (TextNode) p.childNode(0);
assertEquals("Hello", text.getWholeText());
}
@Test public void parsesUnterminatedComments() {
String html = "<p>Hello<!-- <tr><td>";
Document doc = Jsoup.parse(html);
Element p = doc.getElementsByTag("p").get(0);
assertEquals("Hello", p.text());
TextNode text = (TextNode) p.childNode(0);
assertEquals("Hello", text.getWholeText());
Comment comment = (Comment) p.childNode(1);
assertEquals(" <tr><td>", comment.getData());
}
@Test public void parsesUnterminatedTag() {
String h1 = "<p";
Document doc = Jsoup.parse(h1);
assertEquals(1, doc.getElementsByTag("p").size());
String h2 = "<div id=1<p id='2'";
doc = Jsoup.parse(h2);
Element d = doc.getElementById("1");
assertEquals(1, d.children().size());
Element p = doc.getElementById("2");
assertNotNull(p);
}
@Test public void parsesUnterminatedAttribute() {
String h1 = "<p id=\"foo";
Document doc = Jsoup.parse(h1);
Element p = doc.getElementById("foo");
assertNotNull(p);
assertEquals("p", p.tagName());
}
@Test public void parsesUnterminatedTextarea() {
Document doc = Jsoup.parse("<body><p><textarea>one<p>two");
Element t = doc.select("textarea").first();
assertEquals("one<p>two", t.text());
}
@Test public void parsesUnterminatedOption() {
Document doc = Jsoup.parse("<body><p><select><option>One<option>Two</p><p>Three</p>");
Elements options = doc.select("option");
assertEquals(2, options.size());
assertEquals("One", options.first().text());
assertEquals("Two", options.last().text());
}
@Test public void testSpaceAfterTag() {
Document doc = Jsoup.parse("<div > <a name=\"top\"></a ><p id=1 >Hello</p></div>");
assertEquals("<div> <a name=\"top\"></a><p id=\"1\">Hello</p></div>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void createsDocumentStructure() {
String html = "<meta name=keywords /><link rel=stylesheet /><title>jsoup</title><p>Hello world</p>";
Document doc = Jsoup.parse(html);
Element head = doc.head();
Element body = doc.body();
assertEquals(1, doc.children().size()); // root node: contains html node
assertEquals(2, doc.child(0).children().size()); // html node: head and body
assertEquals(3, head.children().size());
assertEquals(1, body.children().size());
assertEquals("keywords", head.getElementsByTag("meta").get(0).attr("name"));
assertEquals(0, body.getElementsByTag("meta").size());
assertEquals("jsoup", doc.title());
assertEquals("Hello world", body.text());
assertEquals("Hello world", body.children().get(0).text());
}
@Test public void createsStructureFromBodySnippet() {
// the bar baz stuff naturally goes into the body, but the 'foo' goes into root, and the normalisation routine
// needs to move into the start of the body
String html = "foo <b>bar</b> baz";
Document doc = Jsoup.parse(html);
assertEquals ("foo bar baz", doc.text());
}
@Test public void handlesEscapedData() {
String html = "<div title='Surf & Turf'>Reef & Beef</div>";
Document doc = Jsoup.parse(html);
Element div = doc.getElementsByTag("div").get(0);
assertEquals("Surf & Turf", div.attr("title"));
assertEquals("Reef & Beef", div.text());
}
@Test public void handlesDataOnlyTags() {
String t = "<style>font-family: bold</style>";
List<Element> tels = Jsoup.parse(t).getElementsByTag("style");
assertEquals("font-family: bold", tels.get(0).data());
assertEquals("", tels.get(0).text());
String s = "<p>Hello</p><script>Nope</script><p>There</p>";
Document doc = Jsoup.parse(s);
assertEquals("Hello There", doc.text());
assertEquals("Nope", doc.data());
}
@Test public void handlesTextAfterData() {
String h = "<html><body>pre <script>inner</script> aft</body></html>";
Document doc = Jsoup.parse(h);
assertEquals("<html><head></head><body>pre <script>inner</script> aft</body></html>", TextUtil.stripNewlines(doc.html()));
}
@Test public void handlesTextArea() {
Document doc = Jsoup.parse("<textarea>Hello</textarea>");
Elements els = doc.select("textarea");
assertEquals("Hello", els.text());
assertEquals("Hello", els.val());
}
@Test public void createsImplicitLists() {
String h = "<li>Point one<li>Point two";
Document doc = Jsoup.parse(h);
Elements ol = doc.select("ul"); // should have created a default ul.
assertEquals(1, ol.size());
assertEquals(2, ol.get(0).children().size());
// no fiddling with non-implicit lists
String h2 = "<ol><li><p>Point the first<li><p>Point the second";
Document doc2 = Jsoup.parse(h2);
assertEquals(0, doc2.select("ul").size());
assertEquals(1, doc2.select("ol").size());
assertEquals(2, doc2.select("ol li").size());
assertEquals(2, doc2.select("ol li p").size());
assertEquals(1, doc2.select("ol li").get(0).children().size()); // one p in first li
}
@Test public void createsImplicitTable() {
String h = "<td>Hello<td><p>There<p>now";
Document doc = Jsoup.parse(h);
assertEquals("<table><tbody><tr><td>Hello</td><td><p>There</p><p>now</p></td></tr></tbody></table>", TextUtil.stripNewlines(doc.body().html()));
// <tbody> is introduced if no implicitly creating table, but allows tr to be directly under table
}
@Test public void handlesNestedImplicitTable() {
Document doc = Jsoup.parse("<table><td>1</td></tr> <td>2</td></tr> <td> <table><td>3</td> <td>4</td></table> <tr><td>5</table>");
assertEquals("<table><tr><td>1</td></tr> <tr><td>2</td></tr> <tr><td> <table><tr><td>3</td> <td>4</td></tr></table> </td></tr><tr><td>5</td></tr></table>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void handlesWhatWgExpensesTableExample() {
// http://www.whatwg.org/specs/web-apps/current-work/multipage/tabular-data.html#examples-0
Document doc = Jsoup.parse("<table> <colgroup> <col> <colgroup> <col> <col> <col> <thead> <tr> <th> <th>2008 <th>2007 <th>2006 <tbody> <tr> <th scope=rowgroup> Research and development <td> $ 1,109 <td> $ 782 <td> $ 712 <tr> <th scope=row> Percentage of net sales <td> 3.4% <td> 3.3% <td> 3.7% <tbody> <tr> <th scope=rowgroup> Selling, general, and administrative <td> $ 3,761 <td> $ 2,963 <td> $ 2,433 <tr> <th scope=row> Percentage of net sales <td> 11.6% <td> 12.3% <td> 12.6% </table>");
assertEquals("<table> <colgroup> <col /> </colgroup><colgroup> <col /> <col /> <col /> </colgroup><thead> <tr> <th> </th><th>2008 </th><th>2007 </th><th>2006 </th></tr></thead><tbody> <tr> <th scope=\"rowgroup\">Research and development </th><td>$ 1,109 </td><td>$ 782 </td><td>$ 712 </td></tr><tr> <th scope=\"row\">Percentage of net sales </th><td>3.4% </td><td>3.3% </td><td>3.7% </td></tr></tbody><tbody> <tr> <th scope=\"rowgroup\">Selling, general, and administrative </th><td>$ 3,761 </td><td>$ 2,963 </td><td>$ 2,433 </td></tr><tr> <th scope=\"row\">Percentage of net sales </th><td>11.6% </td><td>12.3% </td><td>12.6% </td></tr></tbody></table>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void handlesTbodyTable() {
Document doc = Jsoup.parse("<html><head></head><body><table><tbody><tr><td>aaa</td><td>bbb</td></tr></tbody></table></body></html>");
assertEquals("<table><tbody><tr><td>aaa</td><td>bbb</td></tr></tbody></table>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void handlesImplicitCaptionClose() {
Document doc = Jsoup.parse("<table><caption>A caption<td>One<td>Two");
assertEquals("<table><caption>A caption</caption><tr><td>One</td><td>Two</td></tr></table>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void handlesBaseTags() {
String h = "<a href=1>#</a><base href='/2/'><a href='3'>#</a><base href='http://bar'><a href=4>#</a>";
Document doc = Jsoup.parse(h, "http://foo/");
assertEquals("http://bar", doc.baseUri()); // gets updated as base changes, so doc.createElement has latest.
Elements anchors = doc.getElementsByTag("a");
assertEquals(3, anchors.size());
assertEquals("http://foo/", anchors.get(0).baseUri());
assertEquals("http://foo/2/", anchors.get(1).baseUri());
assertEquals("http://bar", anchors.get(2).baseUri());
assertEquals("http://foo/1", anchors.get(0).absUrl("href"));
assertEquals("http://foo/2/3", anchors.get(1).absUrl("href"));
assertEquals("http://bar/4", anchors.get(2).absUrl("href"));
}
@Test public void handlesCdata() {
String h = "<div id=1><![CData[<html>\n<foo><&]]></div>"; // "cdata" insensitive. the & in there should remain literal
Document doc = Jsoup.parse(h);
Element div = doc.getElementById("1");
assertEquals("<html> <foo><&", div.text());
assertEquals(0, div.children().size());
assertEquals(1, div.childNodes().size()); // no elements, one text node
}
@Test public void handlesInvalidStartTags() {
String h = "<div>Hello < There <&></div>"; // parse to <div {#text=Hello < There <&>}>
Document doc = Jsoup.parse(h);
assertEquals("Hello < There <&>", doc.select("div").first().text());
}
@Test public void handlesUnknownTags() {
String h = "<div><foo title=bar>Hello<foo title=qux>there</foo></div>";
Document doc = Jsoup.parse(h);
Elements foos = doc.select("foo");
assertEquals(2, foos.size());
assertEquals("bar", foos.first().attr("title"));
assertEquals("qux", foos.last().attr("title"));
assertEquals("there", foos.last().text());
}
@Test public void handlesUnknownInlineTags() {
String h = "<p><cust>Test</cust></p><p><cust><cust>Test</cust></cust></p>";
Document doc = Jsoup.parseBodyFragment(h);
String out = doc.body().html();
assertEquals(h, TextUtil.stripNewlines(out));
}
@Test public void handlesUnknownNamespaceTags() {
String h = "<foo:bar id=1/><abc:def id=2>Foo<p>Hello</abc:def><foo:bar>There</foo:bar>";
Document doc = Jsoup.parse(h);
assertEquals("<foo:bar id=\"1\" /><abc:def id=\"2\">Foo<p>Hello</p></abc:def><foo:bar>There</foo:bar>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void handlesEmptyBlocks() {
String h = "<div id=1/><div id=2><img /></div>";
Document doc = Jsoup.parse(h);
Element div1 = doc.getElementById("1");
assertTrue(div1.children().isEmpty());
}
@Test public void handlesMultiClosingBody() {
String h = "<body><p>Hello</body><p>there</p></body></body></html><p>now";
Document doc = Jsoup.parse(h);
assertEquals(3, doc.select("p").size());
assertEquals(3, doc.body().children().size());
}
@Test public void handlesUnclosedDefinitionLists() {
String h = "<dt>Foo<dd>Bar<dt>Qux<dd>Zug";
Document doc = Jsoup.parse(h);
assertEquals(4, doc.body().getElementsByTag("dl").first().children().size());
Elements dts = doc.select("dt");
assertEquals(2, dts.size());
assertEquals("Zug", dts.get(1).nextElementSibling().text());
}
@Test public void handlesBlocksInDefinitions() {
// per the spec, dt and dd are inline, but in practise are block
String h = "<dl><dt><div id=1>Term</div></dt><dd><div id=2>Def</div></dd></dl>";
Document doc = Jsoup.parse(h);
assertEquals("dt", doc.select("#1").first().parent().tagName());
assertEquals("dd", doc.select("#2").first().parent().tagName());
assertEquals("<dl><dt><div id=\"1\">Term</div></dt><dd><div id=\"2\">Def</div></dd></dl>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void handlesFrames() {
String h = "<html><head><script></script><noscript></noscript></head><frameset><frame src=foo></frame><frame src=foo></frameset></html>";
Document doc = Jsoup.parse(h);
assertEquals("<html><head><script></script><noscript></noscript></head><frameset><frame src=\"foo\" /><frame src=\"foo\" /></frameset><body></body></html>",
TextUtil.stripNewlines(doc.html()));
}
@Test public void handlesJavadocFont() {
String h = "<TD BGCOLOR=\"#EEEEFF\" CLASS=\"NavBarCell1\"> <A HREF=\"deprecated-list.html\"><FONT CLASS=\"NavBarFont1\"><B>Deprecated</B></FONT></A> </TD>";
Document doc = Jsoup.parse(h);
Element a = doc.select("a").first();
assertEquals("Deprecated", a.text());
assertEquals("font", a.child(0).tagName());
assertEquals("b", a.child(0).child(0).tagName());
}
@Test public void handlesBaseWithoutHref() {
String h = "<head><base target='_blank'></head><body><a href=/foo>Test</a></body>";
Document doc = Jsoup.parse(h, "http://example.com/");
Element a = doc.select("a").first();
assertEquals("/foo", a.attr("href"));
assertEquals("http://example.com/foo", a.attr("abs:href"));
}
@Test public void normalisesDocument() {
String h = "<!doctype html>One<html>Two<head>Three<link></head>Four<body>Five </body>Six </html>Seven ";
Document doc = Jsoup.parse(h);
assertEquals("<!doctype html><html><head><link /></head><body>One Two Four Three Five Six Seven </body></html>",
TextUtil.stripNewlines(doc.html())); // is spaced OK if not newline & space stripped
}
@Test public void normalisesEmptyDocument() {
Document doc = Jsoup.parse("");
assertEquals("<html><head></head><body></body></html>",TextUtil.stripNewlines(doc.html()));
}
@Test public void normalisesHeadlessBody() {
Document doc = Jsoup.parse("<html><body><span class=\"foo\">bar</span>");
assertEquals("<html><head></head><body><span class=\"foo\">bar</span></body></html>",
TextUtil.stripNewlines(doc.html()));
}
@Test public void findsCharsetInMalformedMeta() {
String h = "<meta http-equiv=Content-Type content=text/html; charset=gb2312>";
// example cited for reason of html5's <meta charset> element
Document doc = Jsoup.parse(h);
assertEquals("gb2312", doc.select("meta").attr("charset"));
}
@Test public void testHgroup() {
Document doc = Jsoup.parse("<h1>Hello <h2>There <hgroup><h1>Another<h2>headline</hgroup> <hgroup><h1>More</h1><p>stuff</p></hgroup>");
assertEquals("<h1>Hello </h1><h2>There </h2><hgroup><h1>Another</h1><h2>headline</h2></hgroup> <hgroup><h1>More</h1></hgroup><p>stuff</p>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void testRelaxedTags() {
Document doc = Jsoup.parse("<abc_def id=1>Hello</abc_def> <abc-def>There</abc-def>");
assertEquals("<abc_def id=\"1\">Hello</abc_def> <abc-def>There</abc-def>", TextUtil.stripNewlines(doc.body().html()));
}
} | // You are a professional Java test case writer, please create a test case named `parsesQuiteRoughAttributes` for the issue `Jsoup-32`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Jsoup-32
//
// ## Issue-Title:
// StringIndexOutOfBoundsException when testing whether String content is valid HTML
//
// ## Issue-Description:
// If I try to parse a tag with an equals sign (an empty attribute) but without any single or double quotes around an attribute value, then I get a StringIndexOutOfBoundsException. The stack trace is pasted below.
//
//
// An example String would be "<a =a"
//
//
// The following JUnit test case should not throw a StringIndexOutOfBoundsException:
//
//
// import static org.junit.Assert.assertTrue;
//
// import org.jsoup.Jsoup;
//
// import org.jsoup.safety.Whitelist;
//
// import org.junit.Test;
//
// public class BadAttributeTest {
//
// [@test](https://github.com/test)
//
// public void aTagWithABadAttributeIsValid() throws Exception {
//
// assertTrue(Jsoup.isValid("<a =a", Whitelist.relaxed()));
//
// }
//
// }
//
//
// java.lang.StringIndexOutOfBoundsException: String index out of range: 13
//
// at java.lang.String.charAt(String.java:686)
//
// at org.jsoup.parser.TokenQueue.consume(TokenQueue.java:130)
//
// at org.jsoup.parser.Parser.parseAttribute(Parser.java:207)
//
// at org.jsoup.parser.Parser.parseStartTag(Parser.java:142)
//
// at org.jsoup.parser.Parser.parse(Parser.java:91)
//
// at org.jsoup.parser.Parser.parseBodyFragment(Parser.java:64)
//
// at org.jsoup.Jsoup.parseBodyFragment(Jsoup.java:99)
//
// at org.jsoup.Jsoup.isValid(Jsoup.java:155)
//
//
//
//
@Test public void parsesQuiteRoughAttributes() {
| 52 | 5 | 42 | src/test/java/org/jsoup/parser/ParserTest.java | src/test/java | ```markdown
## Issue-ID: Jsoup-32
## Issue-Title:
StringIndexOutOfBoundsException when testing whether String content is valid HTML
## Issue-Description:
If I try to parse a tag with an equals sign (an empty attribute) but without any single or double quotes around an attribute value, then I get a StringIndexOutOfBoundsException. The stack trace is pasted below.
An example String would be "<a =a"
The following JUnit test case should not throw a StringIndexOutOfBoundsException:
import static org.junit.Assert.assertTrue;
import org.jsoup.Jsoup;
import org.jsoup.safety.Whitelist;
import org.junit.Test;
public class BadAttributeTest {
[@test](https://github.com/test)
public void aTagWithABadAttributeIsValid() throws Exception {
assertTrue(Jsoup.isValid("<a =a", Whitelist.relaxed()));
}
}
java.lang.StringIndexOutOfBoundsException: String index out of range: 13
at java.lang.String.charAt(String.java:686)
at org.jsoup.parser.TokenQueue.consume(TokenQueue.java:130)
at org.jsoup.parser.Parser.parseAttribute(Parser.java:207)
at org.jsoup.parser.Parser.parseStartTag(Parser.java:142)
at org.jsoup.parser.Parser.parse(Parser.java:91)
at org.jsoup.parser.Parser.parseBodyFragment(Parser.java:64)
at org.jsoup.Jsoup.parseBodyFragment(Jsoup.java:99)
at org.jsoup.Jsoup.isValid(Jsoup.java:155)
```
You are a professional Java test case writer, please create a test case named `parsesQuiteRoughAttributes` for the issue `Jsoup-32`, utilizing the provided issue report information and the following function signature.
```java
@Test public void parsesQuiteRoughAttributes() {
```
| 42 | [
"org.jsoup.parser.Parser"
] | 7e2665b68e2145c6cf252864e46a6f91fe6c280939237a5f920ccd31ae0b5af6 | @Test public void parsesQuiteRoughAttributes() | // You are a professional Java test case writer, please create a test case named `parsesQuiteRoughAttributes` for the issue `Jsoup-32`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Jsoup-32
//
// ## Issue-Title:
// StringIndexOutOfBoundsException when testing whether String content is valid HTML
//
// ## Issue-Description:
// If I try to parse a tag with an equals sign (an empty attribute) but without any single or double quotes around an attribute value, then I get a StringIndexOutOfBoundsException. The stack trace is pasted below.
//
//
// An example String would be "<a =a"
//
//
// The following JUnit test case should not throw a StringIndexOutOfBoundsException:
//
//
// import static org.junit.Assert.assertTrue;
//
// import org.jsoup.Jsoup;
//
// import org.jsoup.safety.Whitelist;
//
// import org.junit.Test;
//
// public class BadAttributeTest {
//
// [@test](https://github.com/test)
//
// public void aTagWithABadAttributeIsValid() throws Exception {
//
// assertTrue(Jsoup.isValid("<a =a", Whitelist.relaxed()));
//
// }
//
// }
//
//
// java.lang.StringIndexOutOfBoundsException: String index out of range: 13
//
// at java.lang.String.charAt(String.java:686)
//
// at org.jsoup.parser.TokenQueue.consume(TokenQueue.java:130)
//
// at org.jsoup.parser.Parser.parseAttribute(Parser.java:207)
//
// at org.jsoup.parser.Parser.parseStartTag(Parser.java:142)
//
// at org.jsoup.parser.Parser.parse(Parser.java:91)
//
// at org.jsoup.parser.Parser.parseBodyFragment(Parser.java:64)
//
// at org.jsoup.Jsoup.parseBodyFragment(Jsoup.java:99)
//
// at org.jsoup.Jsoup.isValid(Jsoup.java:155)
//
//
//
//
| Jsoup | package org.jsoup.parser;
import org.jsoup.Jsoup;
import org.jsoup.TextUtil;
import org.jsoup.nodes.Comment;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.nodes.TextNode;
import org.jsoup.select.Elements;
import org.junit.Test;
import java.util.List;
import static org.junit.Assert.*;
/**
Tests for the Parser
@author Jonathan Hedley, [email protected] */
public class ParserTest {
@Test public void parsesSimpleDocument() {
String html = "<html><head><title>First!</title></head><body><p>First post! <img src=\"foo.png\" /></p></body></html>";
Document doc = Jsoup.parse(html);
// need a better way to verify these:
Element p = doc.body().child(0);
assertEquals("p", p.tagName());
Element img = p.child(0);
assertEquals("foo.png", img.attr("src"));
assertEquals("img", img.tagName());
}
@Test public void parsesRoughAttributes() {
String html = "<html><head><title>First!</title></head><body><p class=\"foo > bar\">First post! <img src=\"foo.png\" /></p></body></html>";
Document doc = Jsoup.parse(html);
// need a better way to verify these:
Element p = doc.body().child(0);
assertEquals("p", p.tagName());
assertEquals("foo > bar", p.attr("class"));
}
@Test public void parsesQuiteRoughAttributes() {
String html = "<p =a>One<a =a";
Document doc = Jsoup.parse(html);
assertEquals("<p>One<a></a></p>", doc.body().html());
doc = Jsoup.parse("<p .....");
assertEquals("<p></p>", doc.body().html());
doc = Jsoup.parse("<p .....<p!!");
assertEquals("<p></p>\n<p></p>", doc.body().html());
}
@Test public void parsesComments() {
String html = "<html><head></head><body><img src=foo><!-- <table><tr><td></table> --><p>Hello</p></body></html>";
Document doc = Jsoup.parse(html);
Element body = doc.body();
Comment comment = (Comment) body.childNode(1); // comment should not be sub of img, as it's an empty tag
assertEquals(" <table><tr><td></table> ", comment.getData());
Element p = body.child(1);
TextNode text = (TextNode) p.childNode(0);
assertEquals("Hello", text.getWholeText());
}
@Test public void parsesUnterminatedComments() {
String html = "<p>Hello<!-- <tr><td>";
Document doc = Jsoup.parse(html);
Element p = doc.getElementsByTag("p").get(0);
assertEquals("Hello", p.text());
TextNode text = (TextNode) p.childNode(0);
assertEquals("Hello", text.getWholeText());
Comment comment = (Comment) p.childNode(1);
assertEquals(" <tr><td>", comment.getData());
}
@Test public void parsesUnterminatedTag() {
String h1 = "<p";
Document doc = Jsoup.parse(h1);
assertEquals(1, doc.getElementsByTag("p").size());
String h2 = "<div id=1<p id='2'";
doc = Jsoup.parse(h2);
Element d = doc.getElementById("1");
assertEquals(1, d.children().size());
Element p = doc.getElementById("2");
assertNotNull(p);
}
@Test public void parsesUnterminatedAttribute() {
String h1 = "<p id=\"foo";
Document doc = Jsoup.parse(h1);
Element p = doc.getElementById("foo");
assertNotNull(p);
assertEquals("p", p.tagName());
}
@Test public void parsesUnterminatedTextarea() {
Document doc = Jsoup.parse("<body><p><textarea>one<p>two");
Element t = doc.select("textarea").first();
assertEquals("one<p>two", t.text());
}
@Test public void parsesUnterminatedOption() {
Document doc = Jsoup.parse("<body><p><select><option>One<option>Two</p><p>Three</p>");
Elements options = doc.select("option");
assertEquals(2, options.size());
assertEquals("One", options.first().text());
assertEquals("Two", options.last().text());
}
@Test public void testSpaceAfterTag() {
Document doc = Jsoup.parse("<div > <a name=\"top\"></a ><p id=1 >Hello</p></div>");
assertEquals("<div> <a name=\"top\"></a><p id=\"1\">Hello</p></div>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void createsDocumentStructure() {
String html = "<meta name=keywords /><link rel=stylesheet /><title>jsoup</title><p>Hello world</p>";
Document doc = Jsoup.parse(html);
Element head = doc.head();
Element body = doc.body();
assertEquals(1, doc.children().size()); // root node: contains html node
assertEquals(2, doc.child(0).children().size()); // html node: head and body
assertEquals(3, head.children().size());
assertEquals(1, body.children().size());
assertEquals("keywords", head.getElementsByTag("meta").get(0).attr("name"));
assertEquals(0, body.getElementsByTag("meta").size());
assertEquals("jsoup", doc.title());
assertEquals("Hello world", body.text());
assertEquals("Hello world", body.children().get(0).text());
}
@Test public void createsStructureFromBodySnippet() {
// the bar baz stuff naturally goes into the body, but the 'foo' goes into root, and the normalisation routine
// needs to move into the start of the body
String html = "foo <b>bar</b> baz";
Document doc = Jsoup.parse(html);
assertEquals ("foo bar baz", doc.text());
}
@Test public void handlesEscapedData() {
String html = "<div title='Surf & Turf'>Reef & Beef</div>";
Document doc = Jsoup.parse(html);
Element div = doc.getElementsByTag("div").get(0);
assertEquals("Surf & Turf", div.attr("title"));
assertEquals("Reef & Beef", div.text());
}
@Test public void handlesDataOnlyTags() {
String t = "<style>font-family: bold</style>";
List<Element> tels = Jsoup.parse(t).getElementsByTag("style");
assertEquals("font-family: bold", tels.get(0).data());
assertEquals("", tels.get(0).text());
String s = "<p>Hello</p><script>Nope</script><p>There</p>";
Document doc = Jsoup.parse(s);
assertEquals("Hello There", doc.text());
assertEquals("Nope", doc.data());
}
@Test public void handlesTextAfterData() {
String h = "<html><body>pre <script>inner</script> aft</body></html>";
Document doc = Jsoup.parse(h);
assertEquals("<html><head></head><body>pre <script>inner</script> aft</body></html>", TextUtil.stripNewlines(doc.html()));
}
@Test public void handlesTextArea() {
Document doc = Jsoup.parse("<textarea>Hello</textarea>");
Elements els = doc.select("textarea");
assertEquals("Hello", els.text());
assertEquals("Hello", els.val());
}
@Test public void createsImplicitLists() {
String h = "<li>Point one<li>Point two";
Document doc = Jsoup.parse(h);
Elements ol = doc.select("ul"); // should have created a default ul.
assertEquals(1, ol.size());
assertEquals(2, ol.get(0).children().size());
// no fiddling with non-implicit lists
String h2 = "<ol><li><p>Point the first<li><p>Point the second";
Document doc2 = Jsoup.parse(h2);
assertEquals(0, doc2.select("ul").size());
assertEquals(1, doc2.select("ol").size());
assertEquals(2, doc2.select("ol li").size());
assertEquals(2, doc2.select("ol li p").size());
assertEquals(1, doc2.select("ol li").get(0).children().size()); // one p in first li
}
@Test public void createsImplicitTable() {
String h = "<td>Hello<td><p>There<p>now";
Document doc = Jsoup.parse(h);
assertEquals("<table><tbody><tr><td>Hello</td><td><p>There</p><p>now</p></td></tr></tbody></table>", TextUtil.stripNewlines(doc.body().html()));
// <tbody> is introduced if no implicitly creating table, but allows tr to be directly under table
}
@Test public void handlesNestedImplicitTable() {
Document doc = Jsoup.parse("<table><td>1</td></tr> <td>2</td></tr> <td> <table><td>3</td> <td>4</td></table> <tr><td>5</table>");
assertEquals("<table><tr><td>1</td></tr> <tr><td>2</td></tr> <tr><td> <table><tr><td>3</td> <td>4</td></tr></table> </td></tr><tr><td>5</td></tr></table>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void handlesWhatWgExpensesTableExample() {
// http://www.whatwg.org/specs/web-apps/current-work/multipage/tabular-data.html#examples-0
Document doc = Jsoup.parse("<table> <colgroup> <col> <colgroup> <col> <col> <col> <thead> <tr> <th> <th>2008 <th>2007 <th>2006 <tbody> <tr> <th scope=rowgroup> Research and development <td> $ 1,109 <td> $ 782 <td> $ 712 <tr> <th scope=row> Percentage of net sales <td> 3.4% <td> 3.3% <td> 3.7% <tbody> <tr> <th scope=rowgroup> Selling, general, and administrative <td> $ 3,761 <td> $ 2,963 <td> $ 2,433 <tr> <th scope=row> Percentage of net sales <td> 11.6% <td> 12.3% <td> 12.6% </table>");
assertEquals("<table> <colgroup> <col /> </colgroup><colgroup> <col /> <col /> <col /> </colgroup><thead> <tr> <th> </th><th>2008 </th><th>2007 </th><th>2006 </th></tr></thead><tbody> <tr> <th scope=\"rowgroup\">Research and development </th><td>$ 1,109 </td><td>$ 782 </td><td>$ 712 </td></tr><tr> <th scope=\"row\">Percentage of net sales </th><td>3.4% </td><td>3.3% </td><td>3.7% </td></tr></tbody><tbody> <tr> <th scope=\"rowgroup\">Selling, general, and administrative </th><td>$ 3,761 </td><td>$ 2,963 </td><td>$ 2,433 </td></tr><tr> <th scope=\"row\">Percentage of net sales </th><td>11.6% </td><td>12.3% </td><td>12.6% </td></tr></tbody></table>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void handlesTbodyTable() {
Document doc = Jsoup.parse("<html><head></head><body><table><tbody><tr><td>aaa</td><td>bbb</td></tr></tbody></table></body></html>");
assertEquals("<table><tbody><tr><td>aaa</td><td>bbb</td></tr></tbody></table>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void handlesImplicitCaptionClose() {
Document doc = Jsoup.parse("<table><caption>A caption<td>One<td>Two");
assertEquals("<table><caption>A caption</caption><tr><td>One</td><td>Two</td></tr></table>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void handlesBaseTags() {
String h = "<a href=1>#</a><base href='/2/'><a href='3'>#</a><base href='http://bar'><a href=4>#</a>";
Document doc = Jsoup.parse(h, "http://foo/");
assertEquals("http://bar", doc.baseUri()); // gets updated as base changes, so doc.createElement has latest.
Elements anchors = doc.getElementsByTag("a");
assertEquals(3, anchors.size());
assertEquals("http://foo/", anchors.get(0).baseUri());
assertEquals("http://foo/2/", anchors.get(1).baseUri());
assertEquals("http://bar", anchors.get(2).baseUri());
assertEquals("http://foo/1", anchors.get(0).absUrl("href"));
assertEquals("http://foo/2/3", anchors.get(1).absUrl("href"));
assertEquals("http://bar/4", anchors.get(2).absUrl("href"));
}
@Test public void handlesCdata() {
String h = "<div id=1><![CData[<html>\n<foo><&]]></div>"; // "cdata" insensitive. the & in there should remain literal
Document doc = Jsoup.parse(h);
Element div = doc.getElementById("1");
assertEquals("<html> <foo><&", div.text());
assertEquals(0, div.children().size());
assertEquals(1, div.childNodes().size()); // no elements, one text node
}
@Test public void handlesInvalidStartTags() {
String h = "<div>Hello < There <&></div>"; // parse to <div {#text=Hello < There <&>}>
Document doc = Jsoup.parse(h);
assertEquals("Hello < There <&>", doc.select("div").first().text());
}
@Test public void handlesUnknownTags() {
String h = "<div><foo title=bar>Hello<foo title=qux>there</foo></div>";
Document doc = Jsoup.parse(h);
Elements foos = doc.select("foo");
assertEquals(2, foos.size());
assertEquals("bar", foos.first().attr("title"));
assertEquals("qux", foos.last().attr("title"));
assertEquals("there", foos.last().text());
}
@Test public void handlesUnknownInlineTags() {
String h = "<p><cust>Test</cust></p><p><cust><cust>Test</cust></cust></p>";
Document doc = Jsoup.parseBodyFragment(h);
String out = doc.body().html();
assertEquals(h, TextUtil.stripNewlines(out));
}
@Test public void handlesUnknownNamespaceTags() {
String h = "<foo:bar id=1/><abc:def id=2>Foo<p>Hello</abc:def><foo:bar>There</foo:bar>";
Document doc = Jsoup.parse(h);
assertEquals("<foo:bar id=\"1\" /><abc:def id=\"2\">Foo<p>Hello</p></abc:def><foo:bar>There</foo:bar>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void handlesEmptyBlocks() {
String h = "<div id=1/><div id=2><img /></div>";
Document doc = Jsoup.parse(h);
Element div1 = doc.getElementById("1");
assertTrue(div1.children().isEmpty());
}
@Test public void handlesMultiClosingBody() {
String h = "<body><p>Hello</body><p>there</p></body></body></html><p>now";
Document doc = Jsoup.parse(h);
assertEquals(3, doc.select("p").size());
assertEquals(3, doc.body().children().size());
}
@Test public void handlesUnclosedDefinitionLists() {
String h = "<dt>Foo<dd>Bar<dt>Qux<dd>Zug";
Document doc = Jsoup.parse(h);
assertEquals(4, doc.body().getElementsByTag("dl").first().children().size());
Elements dts = doc.select("dt");
assertEquals(2, dts.size());
assertEquals("Zug", dts.get(1).nextElementSibling().text());
}
@Test public void handlesBlocksInDefinitions() {
// per the spec, dt and dd are inline, but in practise are block
String h = "<dl><dt><div id=1>Term</div></dt><dd><div id=2>Def</div></dd></dl>";
Document doc = Jsoup.parse(h);
assertEquals("dt", doc.select("#1").first().parent().tagName());
assertEquals("dd", doc.select("#2").first().parent().tagName());
assertEquals("<dl><dt><div id=\"1\">Term</div></dt><dd><div id=\"2\">Def</div></dd></dl>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void handlesFrames() {
String h = "<html><head><script></script><noscript></noscript></head><frameset><frame src=foo></frame><frame src=foo></frameset></html>";
Document doc = Jsoup.parse(h);
assertEquals("<html><head><script></script><noscript></noscript></head><frameset><frame src=\"foo\" /><frame src=\"foo\" /></frameset><body></body></html>",
TextUtil.stripNewlines(doc.html()));
}
@Test public void handlesJavadocFont() {
String h = "<TD BGCOLOR=\"#EEEEFF\" CLASS=\"NavBarCell1\"> <A HREF=\"deprecated-list.html\"><FONT CLASS=\"NavBarFont1\"><B>Deprecated</B></FONT></A> </TD>";
Document doc = Jsoup.parse(h);
Element a = doc.select("a").first();
assertEquals("Deprecated", a.text());
assertEquals("font", a.child(0).tagName());
assertEquals("b", a.child(0).child(0).tagName());
}
@Test public void handlesBaseWithoutHref() {
String h = "<head><base target='_blank'></head><body><a href=/foo>Test</a></body>";
Document doc = Jsoup.parse(h, "http://example.com/");
Element a = doc.select("a").first();
assertEquals("/foo", a.attr("href"));
assertEquals("http://example.com/foo", a.attr("abs:href"));
}
@Test public void normalisesDocument() {
String h = "<!doctype html>One<html>Two<head>Three<link></head>Four<body>Five </body>Six </html>Seven ";
Document doc = Jsoup.parse(h);
assertEquals("<!doctype html><html><head><link /></head><body>One Two Four Three Five Six Seven </body></html>",
TextUtil.stripNewlines(doc.html())); // is spaced OK if not newline & space stripped
}
@Test public void normalisesEmptyDocument() {
Document doc = Jsoup.parse("");
assertEquals("<html><head></head><body></body></html>",TextUtil.stripNewlines(doc.html()));
}
@Test public void normalisesHeadlessBody() {
Document doc = Jsoup.parse("<html><body><span class=\"foo\">bar</span>");
assertEquals("<html><head></head><body><span class=\"foo\">bar</span></body></html>",
TextUtil.stripNewlines(doc.html()));
}
@Test public void findsCharsetInMalformedMeta() {
String h = "<meta http-equiv=Content-Type content=text/html; charset=gb2312>";
// example cited for reason of html5's <meta charset> element
Document doc = Jsoup.parse(h);
assertEquals("gb2312", doc.select("meta").attr("charset"));
}
@Test public void testHgroup() {
Document doc = Jsoup.parse("<h1>Hello <h2>There <hgroup><h1>Another<h2>headline</hgroup> <hgroup><h1>More</h1><p>stuff</p></hgroup>");
assertEquals("<h1>Hello </h1><h2>There </h2><hgroup><h1>Another</h1><h2>headline</h2></hgroup> <hgroup><h1>More</h1></hgroup><p>stuff</p>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void testRelaxedTags() {
Document doc = Jsoup.parse("<abc_def id=1>Hello</abc_def> <abc-def>There</abc-def>");
assertEquals("<abc_def id=\"1\">Hello</abc_def> <abc-def>There</abc-def>", TextUtil.stripNewlines(doc.body().html()));
}
} |
||
public void testCustomMapValueDeser735() throws Exception {
String json = "{\"map1\":{\"a\":1},\"map2\":{\"a\":1}}";
TestMapBean735 bean = MAPPER.readValue(json, TestMapBean735.class);
assertEquals(100, bean.map1.get("a").intValue());
assertEquals(1, bean.map2.get("a").intValue());
} | com.fasterxml.jackson.databind.deser.TestCustomDeserializers::testCustomMapValueDeser735 | src/test/java/com/fasterxml/jackson/databind/deser/TestCustomDeserializers.java | 370 | src/test/java/com/fasterxml/jackson/databind/deser/TestCustomDeserializers.java | testCustomMapValueDeser735 | package com.fasterxml.jackson.databind.deser;
import java.io.*;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
import java.util.*;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.core.*;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.*;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
import com.fasterxml.jackson.databind.deser.std.StdDelegatingDeserializer;
import com.fasterxml.jackson.databind.deser.std.StdDeserializer;
import com.fasterxml.jackson.databind.module.SimpleModule;
import com.fasterxml.jackson.databind.util.StdConverter;
/**
* Test to check that customizations work as expected.
*/
@SuppressWarnings("serial")
public class TestCustomDeserializers
extends BaseMapTest
{
/*
/**********************************************************
/* Helper classes
/**********************************************************
*/
static class DummyDeserializer<T>
extends StdDeserializer<T>
{
final T value;
public DummyDeserializer(T v, Class<T> cls) {
super(cls);
value = v;
}
@Override
public T deserialize(JsonParser jp, DeserializationContext ctxt)
throws IOException, JsonProcessingException
{
// need to skip, if structured...
jp.skipChildren();
return value;
}
}
static class TestBeans {
public List<TestBean> beans;
}
static class TestBean {
public CustomBean c;
public String d;
}
@JsonDeserialize(using=CustomBeanDeserializer.class)
static class CustomBean {
protected final int a, b;
public CustomBean(int a, int b) {
this.a = a;
this.b = b;
}
}
static class CustomBeanDeserializer extends JsonDeserializer<CustomBean>
{
@Override
public CustomBean deserialize(JsonParser jp, DeserializationContext ctxt) throws IOException
{
int a = 0, b = 0;
JsonToken t = jp.getCurrentToken();
if (t == JsonToken.START_OBJECT) {
t = jp.nextToken();
} else if (t != JsonToken.FIELD_NAME) {
throw new Error();
}
while(t == JsonToken.FIELD_NAME) {
final String fieldName = jp.getCurrentName();
t = jp.nextToken();
if (t != JsonToken.VALUE_NUMBER_INT) {
throw new JsonParseException("expecting number got "+ t, jp.getCurrentLocation());
}
if (fieldName.equals("a")) {
a = jp.getIntValue();
} else if (fieldName.equals("b")) {
b = jp.getIntValue();
} else {
throw new Error();
}
t = jp.nextToken();
}
return new CustomBean(a, b);
}
}
public static class Immutable {
protected int x, y;
public Immutable(int x0, int y0) {
x = x0;
y = y0;
}
}
// [JACKSON-882]
public static class CustomKey {
private final int id;
public CustomKey(int id) {this.id = id;}
public int getId() { return id; }
}
public static class Model
{
protected final Map<CustomKey, String> map;
@JsonCreator
public Model(@JsonProperty("map") @JsonDeserialize(keyUsing = CustomKeyDeserializer.class) Map<CustomKey, String> map)
{
this.map = new HashMap<CustomKey, String>(map);
}
@JsonProperty
@JsonSerialize(keyUsing = CustomKeySerializer.class)
public Map<CustomKey, String> getMap() {
return map;
}
}
static class CustomKeySerializer extends JsonSerializer<CustomKey> {
@Override
public void serialize(CustomKey value, JsonGenerator jgen, SerializerProvider provider) throws IOException {
jgen.writeFieldName(String.valueOf(value.getId()));
}
}
static class CustomKeyDeserializer extends KeyDeserializer {
@Override
public CustomKey deserializeKey(String key, DeserializationContext ctxt) throws IOException {
return new CustomKey(Integer.valueOf(key));
}
}
// [#375]
@Target({ElementType.FIELD})
@Retention(RetentionPolicy.RUNTIME)
@interface Negative { }
static class Bean375Wrapper {
@Negative
public Bean375Outer value;
}
static class Bean375Outer {
protected Bean375Inner inner;
public Bean375Outer(Bean375Inner v) { inner = v; }
}
static class Bean375Inner {
protected int x;
public Bean375Inner(int x) { this.x = x; }
}
static class Bean375OuterDeserializer extends StdDeserializer<Bean375Outer>
implements ContextualDeserializer
{
protected BeanProperty prop;
protected Bean375OuterDeserializer() { this(null); }
protected Bean375OuterDeserializer(BeanProperty p) {
super(Bean375Outer.class);
prop = p;
}
@Override
public Bean375Outer deserialize(JsonParser p, DeserializationContext ctxt) throws IOException,
JsonProcessingException {
Object ob = ctxt.readPropertyValue(p, prop, Bean375Inner.class);
return new Bean375Outer((Bean375Inner) ob);
}
@Override
public JsonDeserializer<?> createContextual(DeserializationContext ctxt, BeanProperty property)
throws JsonMappingException {
return new Bean375OuterDeserializer(property);
}
}
static class Bean375InnerDeserializer extends StdDeserializer<Bean375Inner>
implements ContextualDeserializer
{
protected boolean negative;
protected Bean375InnerDeserializer() { this(false); }
protected Bean375InnerDeserializer(boolean n) {
super(Bean375Inner.class);
negative = n;
}
@Override
public Bean375Inner deserialize(JsonParser jp, DeserializationContext ctxt)
throws IOException, JsonProcessingException {
int x = jp.getIntValue();
if (negative) {
x = -x;
} else {
x += x;
}
return new Bean375Inner(x);
}
@Override
public JsonDeserializer<?> createContextual(DeserializationContext ctxt, BeanProperty property)
throws JsonMappingException {
if (property != null) {
Negative n = property.getAnnotation(Negative.class);
if (n != null) {
return new Bean375InnerDeserializer(true);
}
}
return this;
}
}
// For [databind#735]
public static class TestMapBean735 {
@JsonDeserialize(contentUsing = CustomDeserializer735.class)
public Map<String, Integer> map1;
public Map<String, Integer> map2;
}
public static class TestListBean735 {
@JsonDeserialize(contentUsing = CustomDeserializer735.class)
public List<Integer> list1;
public List<Integer> list2;
}
public static class CustomDeserializer735 extends StdDeserializer<Integer> {
public CustomDeserializer735() {
super(Integer.class);
}
@Override
public Integer deserialize(JsonParser p, DeserializationContext ctxt) throws IOException {
return 100 * p.getValueAsInt();
}
}
/*
/**********************************************************
/* Unit tests
/**********************************************************
*/
final ObjectMapper MAPPER = objectMapper();
public void testCustomBeanDeserializer() throws Exception
{
String json = "{\"beans\":[{\"c\":{\"a\":10,\"b\":20},\"d\":\"hello, tatu\"}]}";
TestBeans beans = MAPPER.readValue(json, TestBeans.class);
assertNotNull(beans);
List<TestBean> results = beans.beans;
assertNotNull(results);
assertEquals(1, results.size());
TestBean bean = results.get(0);
assertEquals("hello, tatu", bean.d);
CustomBean c = bean.c;
assertNotNull(c);
assertEquals(10, c.a);
assertEquals(20, c.b);
json = "{\"beans\":[{\"c\":{\"b\":3,\"a\":-4},\"d\":\"\"},"
+"{\"d\":\"abc\", \"c\":{\"b\":15}}]}";
beans = MAPPER.readValue(json, TestBeans.class);
assertNotNull(beans);
results = beans.beans;
assertNotNull(results);
assertEquals(2, results.size());
bean = results.get(0);
assertEquals("", bean.d);
c = bean.c;
assertNotNull(c);
assertEquals(-4, c.a);
assertEquals(3, c.b);
bean = results.get(1);
assertEquals("abc", bean.d);
c = bean.c;
assertNotNull(c);
assertEquals(0, c.a);
assertEquals(15, c.b);
}
// [Issue#87]: delegating deserializer
public void testDelegating() throws Exception
{
ObjectMapper mapper = new ObjectMapper();
SimpleModule module = new SimpleModule("test", Version.unknownVersion());
module.addDeserializer(Immutable.class,
new StdDelegatingDeserializer<Immutable>(
new StdConverter<JsonNode, Immutable>() {
@Override
public Immutable convert(JsonNode value)
{
int x = value.path("x").asInt();
int y = value.path("y").asInt();
return new Immutable(x, y);
}
}
));
mapper.registerModule(module);
Immutable imm = mapper.readValue("{\"x\":3,\"y\":7}", Immutable.class);
assertEquals(3, imm.x);
assertEquals(7, imm.y);
}
public void testIssue882() throws Exception
{
Model original = new Model(Collections.singletonMap(new CustomKey(123), "test"));
String json = MAPPER.writeValueAsString(original);
Model deserialized = MAPPER.readValue(json, Model.class);
assertNotNull(deserialized);
assertNotNull(deserialized.map);
assertEquals(1, deserialized.map.size());
}
// [#337]: convenience methods for custom deserializers to use
public void testContextReadValue() throws Exception
{
ObjectMapper mapper = new ObjectMapper();
SimpleModule module = new SimpleModule("test", Version.unknownVersion());
module.addDeserializer(Bean375Outer.class, new Bean375OuterDeserializer());
module.addDeserializer(Bean375Inner.class, new Bean375InnerDeserializer());
mapper.registerModule(module);
// First, without property; doubles up value:
Bean375Outer outer = mapper.readValue("13", Bean375Outer.class);
assertEquals(26, outer.inner.x);
// then with property; should find annotation, turn negative
Bean375Wrapper w = mapper.readValue("{\"value\":13}", Bean375Wrapper.class);
assertNotNull(w.value);
assertNotNull(w.value.inner);
assertEquals(-13, w.value.inner.x);
}
// [databind#735]: erroneous application of custom deserializer
public void testCustomMapValueDeser735() throws Exception {
String json = "{\"map1\":{\"a\":1},\"map2\":{\"a\":1}}";
TestMapBean735 bean = MAPPER.readValue(json, TestMapBean735.class);
assertEquals(100, bean.map1.get("a").intValue());
assertEquals(1, bean.map2.get("a").intValue());
}
public void testCustomListValueDeser735() throws Exception {
String json = "{\"list1\":[1],\"list2\":[1]}";
TestListBean735 bean = MAPPER.readValue(json, TestListBean735.class);
assertEquals(100, bean.list1.get(0).intValue());
assertEquals(1, bean.list2.get(0).intValue());
}
} | // You are a professional Java test case writer, please create a test case named `testCustomMapValueDeser735` for the issue `JacksonDatabind-735`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonDatabind-735
//
// ## Issue-Title:
// @JsonDeserialize on Map with contentUsing custom deserializer overwrites default behavior
//
// ## Issue-Description:
// I recently updated from version 2.3.3 to 2.5.1 and encountered a new issue with our custom deserializers. They either seemed to stop working or were active on the wrong fields.
//
// I could narrow it down to some change in version 2.4.4 (2.4.3 is still working for me)
//
//
// I wrote a test to show this behavior. It seems to appear when there a two maps with the same key and value types in a bean, and only one of them has a custom deserializer. The deserializer is then falsely used either for both or none of the maps.
//
//
// This test works for me in version 2.4.3 and fails with higher versions.
//
//
//
// ```
// import static org.junit.Assert.assertEquals;
//
// import java.io.IOException;
// import java.util.Map;
//
// import org.junit.Test;
//
// import com.fasterxml.jackson.annotation.JsonProperty;
// import com.fasterxml.jackson.core.JsonParser;
// import com.fasterxml.jackson.core.JsonProcessingException;
// import com.fasterxml.jackson.databind.DeserializationContext;
// import com.fasterxml.jackson.databind.ObjectMapper;
// import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
// import com.fasterxml.jackson.databind.deser.std.StdDeserializer;
//
// public class DeserializeTest {
//
// @Test
// public void testIt() throws Exception {
// ObjectMapper om = new ObjectMapper();
// String json = "{\"map1\":{\"a\":1},\"map2\":{\"a\":1}}";
// TestBean bean = om.readValue(json.getBytes(), TestBean.class);
//
// assertEquals(100, bean.getMap1().get("a").intValue());
// assertEquals(1, bean.getMap2().get("a").intValue());
// }
//
// public static class TestBean {
//
// @JsonProperty("map1")
// @JsonDeserialize(contentUsing = CustomDeserializer.class)
// Map<String, Integer> map1;
//
// @JsonProperty("map2")
// Map<String, Integer> map2;
//
// public Map<String, Integer> getMap1() {
// return map1;
// }
//
// public void setMap1(Map<String, Integer> map1) {
// this.map1 = map1;
// }
//
// public Map<String, Integer> getMap2() {
// return map2;
// }
//
// public void setMap2(Map<String, Integer> map2) {
// this.map2 = map2;
// }
// }
//
// public static class CustomDeserializer extends StdDeserializer<Integer> {
//
// public CustomDeserializer() {
// super(Integer.class);
// }
//
// @Override
// public Integer deserialize(JsonParser p, DeserializationContext ctxt) throws IOException, JsonProcessingException {
// Integer value = p.readValueAs(Integer.class);
// return value * 100;
// }
//
public void testCustomMapValueDeser735() throws Exception {
| 370 | // [databind#735]: erroneous application of custom deserializer | 12 | 364 | src/test/java/com/fasterxml/jackson/databind/deser/TestCustomDeserializers.java | src/test/java | ```markdown
## Issue-ID: JacksonDatabind-735
## Issue-Title:
@JsonDeserialize on Map with contentUsing custom deserializer overwrites default behavior
## Issue-Description:
I recently updated from version 2.3.3 to 2.5.1 and encountered a new issue with our custom deserializers. They either seemed to stop working or were active on the wrong fields.
I could narrow it down to some change in version 2.4.4 (2.4.3 is still working for me)
I wrote a test to show this behavior. It seems to appear when there a two maps with the same key and value types in a bean, and only one of them has a custom deserializer. The deserializer is then falsely used either for both or none of the maps.
This test works for me in version 2.4.3 and fails with higher versions.
```
import static org.junit.Assert.assertEquals;
import java.io.IOException;
import java.util.Map;
import org.junit.Test;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.DeserializationContext;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.deser.std.StdDeserializer;
public class DeserializeTest {
@Test
public void testIt() throws Exception {
ObjectMapper om = new ObjectMapper();
String json = "{\"map1\":{\"a\":1},\"map2\":{\"a\":1}}";
TestBean bean = om.readValue(json.getBytes(), TestBean.class);
assertEquals(100, bean.getMap1().get("a").intValue());
assertEquals(1, bean.getMap2().get("a").intValue());
}
public static class TestBean {
@JsonProperty("map1")
@JsonDeserialize(contentUsing = CustomDeserializer.class)
Map<String, Integer> map1;
@JsonProperty("map2")
Map<String, Integer> map2;
public Map<String, Integer> getMap1() {
return map1;
}
public void setMap1(Map<String, Integer> map1) {
this.map1 = map1;
}
public Map<String, Integer> getMap2() {
return map2;
}
public void setMap2(Map<String, Integer> map2) {
this.map2 = map2;
}
}
public static class CustomDeserializer extends StdDeserializer<Integer> {
public CustomDeserializer() {
super(Integer.class);
}
@Override
public Integer deserialize(JsonParser p, DeserializationContext ctxt) throws IOException, JsonProcessingException {
Integer value = p.readValueAs(Integer.class);
return value * 100;
}
```
You are a professional Java test case writer, please create a test case named `testCustomMapValueDeser735` for the issue `JacksonDatabind-735`, utilizing the provided issue report information and the following function signature.
```java
public void testCustomMapValueDeser735() throws Exception {
```
| 364 | [
"com.fasterxml.jackson.databind.deser.std.MapDeserializer"
] | 7ecec775c59b200c1c47892ac1aba008f8100ed9c986feca92dceda06d3af47d | public void testCustomMapValueDeser735() throws Exception | // You are a professional Java test case writer, please create a test case named `testCustomMapValueDeser735` for the issue `JacksonDatabind-735`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonDatabind-735
//
// ## Issue-Title:
// @JsonDeserialize on Map with contentUsing custom deserializer overwrites default behavior
//
// ## Issue-Description:
// I recently updated from version 2.3.3 to 2.5.1 and encountered a new issue with our custom deserializers. They either seemed to stop working or were active on the wrong fields.
//
// I could narrow it down to some change in version 2.4.4 (2.4.3 is still working for me)
//
//
// I wrote a test to show this behavior. It seems to appear when there a two maps with the same key and value types in a bean, and only one of them has a custom deserializer. The deserializer is then falsely used either for both or none of the maps.
//
//
// This test works for me in version 2.4.3 and fails with higher versions.
//
//
//
// ```
// import static org.junit.Assert.assertEquals;
//
// import java.io.IOException;
// import java.util.Map;
//
// import org.junit.Test;
//
// import com.fasterxml.jackson.annotation.JsonProperty;
// import com.fasterxml.jackson.core.JsonParser;
// import com.fasterxml.jackson.core.JsonProcessingException;
// import com.fasterxml.jackson.databind.DeserializationContext;
// import com.fasterxml.jackson.databind.ObjectMapper;
// import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
// import com.fasterxml.jackson.databind.deser.std.StdDeserializer;
//
// public class DeserializeTest {
//
// @Test
// public void testIt() throws Exception {
// ObjectMapper om = new ObjectMapper();
// String json = "{\"map1\":{\"a\":1},\"map2\":{\"a\":1}}";
// TestBean bean = om.readValue(json.getBytes(), TestBean.class);
//
// assertEquals(100, bean.getMap1().get("a").intValue());
// assertEquals(1, bean.getMap2().get("a").intValue());
// }
//
// public static class TestBean {
//
// @JsonProperty("map1")
// @JsonDeserialize(contentUsing = CustomDeserializer.class)
// Map<String, Integer> map1;
//
// @JsonProperty("map2")
// Map<String, Integer> map2;
//
// public Map<String, Integer> getMap1() {
// return map1;
// }
//
// public void setMap1(Map<String, Integer> map1) {
// this.map1 = map1;
// }
//
// public Map<String, Integer> getMap2() {
// return map2;
// }
//
// public void setMap2(Map<String, Integer> map2) {
// this.map2 = map2;
// }
// }
//
// public static class CustomDeserializer extends StdDeserializer<Integer> {
//
// public CustomDeserializer() {
// super(Integer.class);
// }
//
// @Override
// public Integer deserialize(JsonParser p, DeserializationContext ctxt) throws IOException, JsonProcessingException {
// Integer value = p.readValueAs(Integer.class);
// return value * 100;
// }
//
| JacksonDatabind | package com.fasterxml.jackson.databind.deser;
import java.io.*;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
import java.util.*;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.core.*;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.*;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
import com.fasterxml.jackson.databind.deser.std.StdDelegatingDeserializer;
import com.fasterxml.jackson.databind.deser.std.StdDeserializer;
import com.fasterxml.jackson.databind.module.SimpleModule;
import com.fasterxml.jackson.databind.util.StdConverter;
/**
* Test to check that customizations work as expected.
*/
@SuppressWarnings("serial")
public class TestCustomDeserializers
extends BaseMapTest
{
/*
/**********************************************************
/* Helper classes
/**********************************************************
*/
static class DummyDeserializer<T>
extends StdDeserializer<T>
{
final T value;
public DummyDeserializer(T v, Class<T> cls) {
super(cls);
value = v;
}
@Override
public T deserialize(JsonParser jp, DeserializationContext ctxt)
throws IOException, JsonProcessingException
{
// need to skip, if structured...
jp.skipChildren();
return value;
}
}
static class TestBeans {
public List<TestBean> beans;
}
static class TestBean {
public CustomBean c;
public String d;
}
@JsonDeserialize(using=CustomBeanDeserializer.class)
static class CustomBean {
protected final int a, b;
public CustomBean(int a, int b) {
this.a = a;
this.b = b;
}
}
static class CustomBeanDeserializer extends JsonDeserializer<CustomBean>
{
@Override
public CustomBean deserialize(JsonParser jp, DeserializationContext ctxt) throws IOException
{
int a = 0, b = 0;
JsonToken t = jp.getCurrentToken();
if (t == JsonToken.START_OBJECT) {
t = jp.nextToken();
} else if (t != JsonToken.FIELD_NAME) {
throw new Error();
}
while(t == JsonToken.FIELD_NAME) {
final String fieldName = jp.getCurrentName();
t = jp.nextToken();
if (t != JsonToken.VALUE_NUMBER_INT) {
throw new JsonParseException("expecting number got "+ t, jp.getCurrentLocation());
}
if (fieldName.equals("a")) {
a = jp.getIntValue();
} else if (fieldName.equals("b")) {
b = jp.getIntValue();
} else {
throw new Error();
}
t = jp.nextToken();
}
return new CustomBean(a, b);
}
}
public static class Immutable {
protected int x, y;
public Immutable(int x0, int y0) {
x = x0;
y = y0;
}
}
// [JACKSON-882]
public static class CustomKey {
private final int id;
public CustomKey(int id) {this.id = id;}
public int getId() { return id; }
}
public static class Model
{
protected final Map<CustomKey, String> map;
@JsonCreator
public Model(@JsonProperty("map") @JsonDeserialize(keyUsing = CustomKeyDeserializer.class) Map<CustomKey, String> map)
{
this.map = new HashMap<CustomKey, String>(map);
}
@JsonProperty
@JsonSerialize(keyUsing = CustomKeySerializer.class)
public Map<CustomKey, String> getMap() {
return map;
}
}
static class CustomKeySerializer extends JsonSerializer<CustomKey> {
@Override
public void serialize(CustomKey value, JsonGenerator jgen, SerializerProvider provider) throws IOException {
jgen.writeFieldName(String.valueOf(value.getId()));
}
}
static class CustomKeyDeserializer extends KeyDeserializer {
@Override
public CustomKey deserializeKey(String key, DeserializationContext ctxt) throws IOException {
return new CustomKey(Integer.valueOf(key));
}
}
// [#375]
@Target({ElementType.FIELD})
@Retention(RetentionPolicy.RUNTIME)
@interface Negative { }
static class Bean375Wrapper {
@Negative
public Bean375Outer value;
}
static class Bean375Outer {
protected Bean375Inner inner;
public Bean375Outer(Bean375Inner v) { inner = v; }
}
static class Bean375Inner {
protected int x;
public Bean375Inner(int x) { this.x = x; }
}
static class Bean375OuterDeserializer extends StdDeserializer<Bean375Outer>
implements ContextualDeserializer
{
protected BeanProperty prop;
protected Bean375OuterDeserializer() { this(null); }
protected Bean375OuterDeserializer(BeanProperty p) {
super(Bean375Outer.class);
prop = p;
}
@Override
public Bean375Outer deserialize(JsonParser p, DeserializationContext ctxt) throws IOException,
JsonProcessingException {
Object ob = ctxt.readPropertyValue(p, prop, Bean375Inner.class);
return new Bean375Outer((Bean375Inner) ob);
}
@Override
public JsonDeserializer<?> createContextual(DeserializationContext ctxt, BeanProperty property)
throws JsonMappingException {
return new Bean375OuterDeserializer(property);
}
}
static class Bean375InnerDeserializer extends StdDeserializer<Bean375Inner>
implements ContextualDeserializer
{
protected boolean negative;
protected Bean375InnerDeserializer() { this(false); }
protected Bean375InnerDeserializer(boolean n) {
super(Bean375Inner.class);
negative = n;
}
@Override
public Bean375Inner deserialize(JsonParser jp, DeserializationContext ctxt)
throws IOException, JsonProcessingException {
int x = jp.getIntValue();
if (negative) {
x = -x;
} else {
x += x;
}
return new Bean375Inner(x);
}
@Override
public JsonDeserializer<?> createContextual(DeserializationContext ctxt, BeanProperty property)
throws JsonMappingException {
if (property != null) {
Negative n = property.getAnnotation(Negative.class);
if (n != null) {
return new Bean375InnerDeserializer(true);
}
}
return this;
}
}
// For [databind#735]
public static class TestMapBean735 {
@JsonDeserialize(contentUsing = CustomDeserializer735.class)
public Map<String, Integer> map1;
public Map<String, Integer> map2;
}
public static class TestListBean735 {
@JsonDeserialize(contentUsing = CustomDeserializer735.class)
public List<Integer> list1;
public List<Integer> list2;
}
public static class CustomDeserializer735 extends StdDeserializer<Integer> {
public CustomDeserializer735() {
super(Integer.class);
}
@Override
public Integer deserialize(JsonParser p, DeserializationContext ctxt) throws IOException {
return 100 * p.getValueAsInt();
}
}
/*
/**********************************************************
/* Unit tests
/**********************************************************
*/
final ObjectMapper MAPPER = objectMapper();
public void testCustomBeanDeserializer() throws Exception
{
String json = "{\"beans\":[{\"c\":{\"a\":10,\"b\":20},\"d\":\"hello, tatu\"}]}";
TestBeans beans = MAPPER.readValue(json, TestBeans.class);
assertNotNull(beans);
List<TestBean> results = beans.beans;
assertNotNull(results);
assertEquals(1, results.size());
TestBean bean = results.get(0);
assertEquals("hello, tatu", bean.d);
CustomBean c = bean.c;
assertNotNull(c);
assertEquals(10, c.a);
assertEquals(20, c.b);
json = "{\"beans\":[{\"c\":{\"b\":3,\"a\":-4},\"d\":\"\"},"
+"{\"d\":\"abc\", \"c\":{\"b\":15}}]}";
beans = MAPPER.readValue(json, TestBeans.class);
assertNotNull(beans);
results = beans.beans;
assertNotNull(results);
assertEquals(2, results.size());
bean = results.get(0);
assertEquals("", bean.d);
c = bean.c;
assertNotNull(c);
assertEquals(-4, c.a);
assertEquals(3, c.b);
bean = results.get(1);
assertEquals("abc", bean.d);
c = bean.c;
assertNotNull(c);
assertEquals(0, c.a);
assertEquals(15, c.b);
}
// [Issue#87]: delegating deserializer
public void testDelegating() throws Exception
{
ObjectMapper mapper = new ObjectMapper();
SimpleModule module = new SimpleModule("test", Version.unknownVersion());
module.addDeserializer(Immutable.class,
new StdDelegatingDeserializer<Immutable>(
new StdConverter<JsonNode, Immutable>() {
@Override
public Immutable convert(JsonNode value)
{
int x = value.path("x").asInt();
int y = value.path("y").asInt();
return new Immutable(x, y);
}
}
));
mapper.registerModule(module);
Immutable imm = mapper.readValue("{\"x\":3,\"y\":7}", Immutable.class);
assertEquals(3, imm.x);
assertEquals(7, imm.y);
}
public void testIssue882() throws Exception
{
Model original = new Model(Collections.singletonMap(new CustomKey(123), "test"));
String json = MAPPER.writeValueAsString(original);
Model deserialized = MAPPER.readValue(json, Model.class);
assertNotNull(deserialized);
assertNotNull(deserialized.map);
assertEquals(1, deserialized.map.size());
}
// [#337]: convenience methods for custom deserializers to use
public void testContextReadValue() throws Exception
{
ObjectMapper mapper = new ObjectMapper();
SimpleModule module = new SimpleModule("test", Version.unknownVersion());
module.addDeserializer(Bean375Outer.class, new Bean375OuterDeserializer());
module.addDeserializer(Bean375Inner.class, new Bean375InnerDeserializer());
mapper.registerModule(module);
// First, without property; doubles up value:
Bean375Outer outer = mapper.readValue("13", Bean375Outer.class);
assertEquals(26, outer.inner.x);
// then with property; should find annotation, turn negative
Bean375Wrapper w = mapper.readValue("{\"value\":13}", Bean375Wrapper.class);
assertNotNull(w.value);
assertNotNull(w.value.inner);
assertEquals(-13, w.value.inner.x);
}
// [databind#735]: erroneous application of custom deserializer
public void testCustomMapValueDeser735() throws Exception {
String json = "{\"map1\":{\"a\":1},\"map2\":{\"a\":1}}";
TestMapBean735 bean = MAPPER.readValue(json, TestMapBean735.class);
assertEquals(100, bean.map1.get("a").intValue());
assertEquals(1, bean.map2.get("a").intValue());
}
public void testCustomListValueDeser735() throws Exception {
String json = "{\"list1\":[1],\"list2\":[1]}";
TestListBean735 bean = MAPPER.readValue(json, TestListBean735.class);
assertEquals(100, bean.list1.get(0).intValue());
assertEquals(1, bean.list2.get(0).intValue());
}
} |
|
public void testIssue284() {
CompilerOptions options = createCompilerOptions();
options.smartNameRemoval = true;
test(options,
"var goog = {};" +
"goog.inherits = function(x, y) {};" +
"var ns = {};" +
"/** @constructor */" +
"ns.PageSelectionModel = function() {};" +
"/** @constructor */" +
"ns.PageSelectionModel.FooEvent = function() {};" +
"/** @constructor */" +
"ns.PageSelectionModel.SelectEvent = function() {};" +
"goog.inherits(ns.PageSelectionModel.ChangeEvent," +
" ns.PageSelectionModel.FooEvent);",
"");
} | com.google.javascript.jscomp.IntegrationTest::testIssue284 | test/com/google/javascript/jscomp/IntegrationTest.java | 1,781 | test/com/google/javascript/jscomp/IntegrationTest.java | testIssue284 | /*
* Copyright 2009 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import com.google.common.base.Joiner;
import com.google.common.collect.ArrayListMultimap;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.Sets;
import com.google.javascript.jscomp.CompilerOptions.LanguageMode;
import com.google.javascript.rhino.Node;
import com.google.javascript.rhino.Token;
import junit.framework.TestCase;
/**
* Tests for {@link PassFactory}.
*
* @author [email protected] (Nick Santos)
*/
public class IntegrationTest extends TestCase {
/** Externs for the test */
private final JSSourceFile[] DEFAULT_EXTERNS = new JSSourceFile[] {
JSSourceFile.fromCode("externs",
"var arguments;\n"
+ "/** @constructor */ function Window() {}\n"
+ "/** @type {string} */ Window.prototype.name;\n"
+ "/** @type {string} */ Window.prototype.offsetWidth;\n"
+ "/** @type {Window} */ var window;\n"
+ "/** @nosideeffects */ function noSideEffects() {}\n"
+ "/** @constructor\n * @nosideeffects */ function Widget() {}\n"
+ "/** @modifies {this} */ Widget.prototype.go = function() {};\n"
+ "/** @return {string} */ var widgetToken = function() {};\n")
};
private JSSourceFile[] externs = DEFAULT_EXTERNS;
private static final String CLOSURE_BOILERPLATE =
"/** @define {boolean} */ var COMPILED = false; var goog = {};" +
"goog.exportSymbol = function() {};";
private static final String CLOSURE_COMPILED =
"var COMPILED = true; var goog$exportSymbol = function() {};";
// The most recently used compiler.
private Compiler lastCompiler;
@Override
public void setUp() {
externs = DEFAULT_EXTERNS;
lastCompiler = null;
}
public void testBug1949424() {
CompilerOptions options = createCompilerOptions();
options.collapseProperties = true;
options.closurePass = true;
test(options, CLOSURE_BOILERPLATE + "goog.provide('FOO'); FOO.bar = 3;",
CLOSURE_COMPILED + "var FOO$bar = 3;");
}
public void testBug1949424_v2() {
CompilerOptions options = createCompilerOptions();
options.collapseProperties = true;
options.closurePass = true;
test(options, CLOSURE_BOILERPLATE + "goog.provide('FOO.BAR'); FOO.BAR = 3;",
CLOSURE_COMPILED + "var FOO$BAR = 3;");
}
public void testBug1956277() {
CompilerOptions options = createCompilerOptions();
options.collapseProperties = true;
options.inlineVariables = true;
test(options, "var CONST = {}; CONST.bar = null;" +
"function f(url) { CONST.bar = url; }",
"var CONST$bar = null; function f(url) { CONST$bar = url; }");
}
public void testBug1962380() {
CompilerOptions options = createCompilerOptions();
options.collapseProperties = true;
options.inlineVariables = true;
options.generateExports = true;
test(options,
CLOSURE_BOILERPLATE + "/** @export */ goog.CONSTANT = 1;" +
"var x = goog.CONSTANT;",
"(function() {})('goog.CONSTANT', 1);" +
"var x = 1;");
}
public void testBug2410122() {
CompilerOptions options = createCompilerOptions();
options.generateExports = true;
options.closurePass = true;
test(options,
"var goog = {};" +
"function F() {}" +
"/** @export */ function G() { goog.base(this); } " +
"goog.inherits(G, F);",
"var goog = {};" +
"function F() {}" +
"function G() { F.call(this); } " +
"goog.inherits(G, F); goog.exportSymbol('G', G);");
}
public void testIssue90() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
options.inlineVariables = true;
options.removeDeadCode = true;
test(options,
"var x; x && alert(1);",
"");
}
public void testClosurePassOff() {
CompilerOptions options = createCompilerOptions();
options.closurePass = false;
testSame(
options,
"var goog = {}; goog.require = function(x) {}; goog.require('foo');");
testSame(
options,
"var goog = {}; goog.getCssName = function(x) {};" +
"goog.getCssName('foo');");
}
public void testClosurePassOn() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
test(
options,
"var goog = {}; goog.require = function(x) {}; goog.require('foo');",
ProcessClosurePrimitives.MISSING_PROVIDE_ERROR);
test(
options,
"/** @define {boolean} */ var COMPILED = false;" +
"var goog = {}; goog.getCssName = function(x) {};" +
"goog.getCssName('foo');",
"var COMPILED = true;" +
"var goog = {}; goog.getCssName = function(x) {};" +
"'foo';");
}
public void testCssNameCheck() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.checkMissingGetCssNameLevel = CheckLevel.ERROR;
options.checkMissingGetCssNameBlacklist = "foo";
test(options, "var x = 'foo';",
CheckMissingGetCssName.MISSING_GETCSSNAME);
}
public void testBug2592659() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.checkTypes = true;
options.checkMissingGetCssNameLevel = CheckLevel.WARNING;
options.checkMissingGetCssNameBlacklist = "foo";
test(options,
"var goog = {};\n" +
"/**\n" +
" * @param {string} className\n" +
" * @param {string=} opt_modifier\n" +
" * @return {string}\n" +
"*/\n" +
"goog.getCssName = function(className, opt_modifier) {}\n" +
"var x = goog.getCssName(123, 'a');",
TypeValidator.TYPE_MISMATCH_WARNING);
}
public void testTypedefBeforeOwner1() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
test(options,
"goog.provide('foo.Bar.Type');\n" +
"goog.provide('foo.Bar');\n" +
"/** @typedef {number} */ foo.Bar.Type;\n" +
"foo.Bar = function() {};",
"var foo = {}; foo.Bar.Type; foo.Bar = function() {};");
}
public void testTypedefBeforeOwner2() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.collapseProperties = true;
test(options,
"goog.provide('foo.Bar.Type');\n" +
"goog.provide('foo.Bar');\n" +
"/** @typedef {number} */ foo.Bar.Type;\n" +
"foo.Bar = function() {};",
"var foo$Bar$Type; var foo$Bar = function() {};");
}
public void testExportedNames() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.variableRenaming = VariableRenamingPolicy.ALL;
test(options,
"/** @define {boolean} */ var COMPILED = false;" +
"var goog = {}; goog.exportSymbol('b', goog);",
"var a = true; var c = {}; c.exportSymbol('b', c);");
test(options,
"/** @define {boolean} */ var COMPILED = false;" +
"var goog = {}; goog.exportSymbol('a', goog);",
"var b = true; var c = {}; c.exportSymbol('a', c);");
}
public void testCheckGlobalThisOn() {
CompilerOptions options = createCompilerOptions();
options.checkSuspiciousCode = true;
options.checkGlobalThisLevel = CheckLevel.ERROR;
test(options, "function f() { this.y = 3; }", CheckGlobalThis.GLOBAL_THIS);
}
public void testSusiciousCodeOff() {
CompilerOptions options = createCompilerOptions();
options.checkSuspiciousCode = false;
options.checkGlobalThisLevel = CheckLevel.ERROR;
test(options, "function f() { this.y = 3; }", CheckGlobalThis.GLOBAL_THIS);
}
public void testCheckGlobalThisOff() {
CompilerOptions options = createCompilerOptions();
options.checkSuspiciousCode = true;
options.checkGlobalThisLevel = CheckLevel.OFF;
testSame(options, "function f() { this.y = 3; }");
}
public void testCheckRequiresAndCheckProvidesOff() {
testSame(createCompilerOptions(), new String[] {
"/** @constructor */ function Foo() {}",
"new Foo();"
});
}
public void testCheckRequiresOn() {
CompilerOptions options = createCompilerOptions();
options.checkRequires = CheckLevel.ERROR;
test(options, new String[] {
"/** @constructor */ function Foo() {}",
"new Foo();"
}, CheckRequiresForConstructors.MISSING_REQUIRE_WARNING);
}
public void testCheckProvidesOn() {
CompilerOptions options = createCompilerOptions();
options.checkProvides = CheckLevel.ERROR;
test(options, new String[] {
"/** @constructor */ function Foo() {}",
"new Foo();"
}, CheckProvides.MISSING_PROVIDE_WARNING);
}
public void testGenerateExportsOff() {
testSame(createCompilerOptions(), "/** @export */ function f() {}");
}
public void testGenerateExportsOn() {
CompilerOptions options = createCompilerOptions();
options.generateExports = true;
test(options, "/** @export */ function f() {}",
"/** @export */ function f() {} goog.exportSymbol('f', f);");
}
public void testExportTestFunctionsOff() {
testSame(createCompilerOptions(), "function testFoo() {}");
}
public void testExportTestFunctionsOn() {
CompilerOptions options = createCompilerOptions();
options.exportTestFunctions = true;
test(options, "function testFoo() {}",
"/** @export */ function testFoo() {}" +
"goog.exportSymbol('testFoo', testFoo);");
}
public void testCheckSymbolsOff() {
CompilerOptions options = createCompilerOptions();
testSame(options, "x = 3;");
}
public void testCheckSymbolsOn() {
CompilerOptions options = createCompilerOptions();
options.checkSymbols = true;
test(options, "x = 3;", VarCheck.UNDEFINED_VAR_ERROR);
}
public void testCheckReferencesOff() {
CompilerOptions options = createCompilerOptions();
testSame(options, "x = 3; var x = 5;");
}
public void testCheckReferencesOn() {
CompilerOptions options = createCompilerOptions();
options.aggressiveVarCheck = CheckLevel.ERROR;
test(options, "x = 3; var x = 5;",
VariableReferenceCheck.UNDECLARED_REFERENCE);
}
public void testInferTypes() {
CompilerOptions options = createCompilerOptions();
options.inferTypes = true;
options.checkTypes = false;
options.closurePass = true;
test(options,
CLOSURE_BOILERPLATE +
"goog.provide('Foo'); /** @enum */ Foo = {a: 3};",
TypeCheck.ENUM_NOT_CONSTANT);
assertTrue(lastCompiler.getErrorManager().getTypedPercent() == 0);
// This does not generate a warning.
test(options, "/** @type {number} */ var n = window.name;",
"var n = window.name;");
assertTrue(lastCompiler.getErrorManager().getTypedPercent() == 0);
}
public void testTypeCheckAndInference() {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
test(options, "/** @type {number} */ var n = window.name;",
TypeValidator.TYPE_MISMATCH_WARNING);
assertTrue(lastCompiler.getErrorManager().getTypedPercent() > 0);
}
public void testTypeNameParser() {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
test(options, "/** @type {n} */ var n = window.name;",
RhinoErrorReporter.TYPE_PARSE_ERROR);
}
// This tests that the TypedScopeCreator is memoized so that it only creates a
// Scope object once for each scope. If, when type inference requests a scope,
// it creates a new one, then multiple JSType objects end up getting created
// for the same local type, and ambiguate will rename the last statement to
// o.a(o.a, o.a), which is bad.
public void testMemoizedTypedScopeCreator() {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
options.ambiguateProperties = true;
options.propertyRenaming = PropertyRenamingPolicy.ALL_UNQUOTED;
test(options, "function someTest() {\n"
+ " /** @constructor */\n"
+ " function Foo() { this.instProp = 3; }\n"
+ " Foo.prototype.protoProp = function(a, b) {};\n"
+ " /** @constructor\n @extends Foo */\n"
+ " function Bar() {}\n"
+ " goog.inherits(Bar, Foo);\n"
+ " var o = new Bar();\n"
+ " o.protoProp(o.protoProp, o.instProp);\n"
+ "}",
"function someTest() {\n"
+ " function Foo() { this.b = 3; }\n"
+ " Foo.prototype.a = function(a, b) {};\n"
+ " function Bar() {}\n"
+ " goog.c(Bar, Foo);\n"
+ " var o = new Bar();\n"
+ " o.a(o.a, o.b);\n"
+ "}");
}
public void testCheckTypes() {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
test(options, "var x = x || {}; x.f = function() {}; x.f(3);",
TypeCheck.WRONG_ARGUMENT_COUNT);
}
public void testReplaceCssNames() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.gatherCssNames = true;
test(options, "/** @define {boolean} */\n"
+ "var COMPILED = false;\n"
+ "goog.setCssNameMapping({'foo':'bar'});\n"
+ "function getCss() {\n"
+ " return goog.getCssName('foo');\n"
+ "}",
"var COMPILED = true;\n"
+ "function getCss() {\n"
+ " return \"bar\";"
+ "}");
assertEquals(
ImmutableMap.of("foo", new Integer(1)),
lastCompiler.getPassConfig().getIntermediateState().cssNames);
}
public void testRemoveClosureAsserts() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
testSame(options,
"var goog = {};"
+ "goog.asserts.assert(goog);");
options.removeClosureAsserts = true;
test(options,
"var goog = {};"
+ "goog.asserts.assert(goog);",
"var goog = {};");
}
public void testDeprecation() {
String code = "/** @deprecated */ function f() { } function g() { f(); }";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.setWarningLevel(DiagnosticGroups.DEPRECATED, CheckLevel.ERROR);
testSame(options, code);
options.checkTypes = true;
test(options, code, CheckAccessControls.DEPRECATED_NAME);
}
public void testVisibility() {
String[] code = {
"/** @private */ function f() { }",
"function g() { f(); }"
};
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.setWarningLevel(DiagnosticGroups.VISIBILITY, CheckLevel.ERROR);
testSame(options, code);
options.checkTypes = true;
test(options, code, CheckAccessControls.BAD_PRIVATE_GLOBAL_ACCESS);
}
public void testUnreachableCode() {
String code = "function f() { return \n 3; }";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.checkUnreachableCode = CheckLevel.ERROR;
test(options, code, CheckUnreachableCode.UNREACHABLE_CODE);
}
public void testMissingReturn() {
String code =
"/** @return {number} */ function f() { if (f) { return 3; } }";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.checkMissingReturn = CheckLevel.ERROR;
testSame(options, code);
options.checkTypes = true;
test(options, code, CheckMissingReturn.MISSING_RETURN_STATEMENT);
}
public void testIdGenerators() {
String code = "function f() {} f('id');";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.idGenerators = Sets.newHashSet("f");
test(options, code, "function f() {} 'a';");
}
public void testOptimizeArgumentsArray() {
String code = "function f() { return arguments[0]; }";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.optimizeArgumentsArray = true;
String argName = "JSCompiler_OptimizeArgumentsArray_p0";
test(options, code,
"function f(" + argName + ") { return " + argName + "; }");
}
public void testOptimizeParameters() {
String code = "function f(a) { return a; } f(true);";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.optimizeParameters = true;
test(options, code, "function f() { var a = true; return a;} f();");
}
public void testOptimizeReturns() {
String code = "function f(a) { return a; } f(true);";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.optimizeReturns = true;
test(options, code, "function f(a) {return;} f(true);");
}
public void testRemoveAbstractMethods() {
String code = CLOSURE_BOILERPLATE +
"var x = {}; x.foo = goog.abstractMethod; x.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.closurePass = true;
options.collapseProperties = true;
test(options, code, CLOSURE_COMPILED + " var x$bar = 3;");
}
public void testCollapseProperties1() {
String code =
"var x = {}; x.FOO = 5; x.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.collapseProperties = true;
test(options, code, "var x$FOO = 5; var x$bar = 3;");
}
public void testCollapseProperties2() {
String code =
"var x = {}; x.FOO = 5; x.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.collapseProperties = true;
options.collapseObjectLiterals = true;
test(options, code, "var x$FOO = 5; var x$bar = 3;");
}
public void testCollapseObjectLiteral1() {
// Verify collapseObjectLiterals does nothing in global scope
String code = "var x = {}; x.FOO = 5; x.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.collapseObjectLiterals = true;
testSame(options, code);
}
public void testCollapseObjectLiteral2() {
String code =
"function f() {var x = {}; x.FOO = 5; x.bar = 3;}";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.collapseObjectLiterals = true;
test(options, code,
"function f(){" +
"var JSCompiler_object_inline_FOO_0;" +
"var JSCompiler_object_inline_bar_1;" +
"JSCompiler_object_inline_FOO_0=5;" +
"JSCompiler_object_inline_bar_1=3}");
}
public void testTightenTypesWithoutTypeCheck() {
CompilerOptions options = createCompilerOptions();
options.tightenTypes = true;
test(options, "", DefaultPassConfig.TIGHTEN_TYPES_WITHOUT_TYPE_CHECK);
}
public void testDisambiguateProperties() {
String code =
"/** @constructor */ function Foo(){} Foo.prototype.bar = 3;" +
"/** @constructor */ function Baz(){} Baz.prototype.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.disambiguateProperties = true;
options.checkTypes = true;
test(options, code,
"function Foo(){} Foo.prototype.Foo_prototype$bar = 3;" +
"function Baz(){} Baz.prototype.Baz_prototype$bar = 3;");
}
public void testMarkPureCalls() {
String testCode = "function foo() {} foo();";
CompilerOptions options = createCompilerOptions();
options.removeDeadCode = true;
testSame(options, testCode);
options.computeFunctionSideEffects = true;
test(options, testCode, "function foo() {}");
}
public void testMarkNoSideEffects() {
String testCode = "noSideEffects();";
CompilerOptions options = createCompilerOptions();
options.removeDeadCode = true;
testSame(options, testCode);
options.markNoSideEffectCalls = true;
test(options, testCode, "");
}
public void testChainedCalls() {
CompilerOptions options = createCompilerOptions();
options.chainCalls = true;
test(
options,
"/** @constructor */ function Foo() {} " +
"Foo.prototype.bar = function() { return this; }; " +
"var f = new Foo();" +
"f.bar(); " +
"f.bar(); ",
"function Foo() {} " +
"Foo.prototype.bar = function() { return this; }; " +
"var f = new Foo();" +
"f.bar().bar();");
}
public void testExtraAnnotationNames() {
CompilerOptions options = createCompilerOptions();
options.setExtraAnnotationNames(Sets.newHashSet("TagA", "TagB"));
test(
options,
"/** @TagA */ var f = new Foo(); /** @TagB */ f.bar();",
"var f = new Foo(); f.bar();");
}
public void testDevirtualizePrototypeMethods() {
CompilerOptions options = createCompilerOptions();
options.devirtualizePrototypeMethods = true;
test(
options,
"/** @constructor */ var Foo = function() {}; " +
"Foo.prototype.bar = function() {};" +
"(new Foo()).bar();",
"var Foo = function() {};" +
"var JSCompiler_StaticMethods_bar = " +
" function(JSCompiler_StaticMethods_bar$self) {};" +
"JSCompiler_StaticMethods_bar(new Foo());");
}
public void testCheckConsts() {
CompilerOptions options = createCompilerOptions();
options.inlineConstantVars = true;
test(options, "var FOO = true; FOO = false",
ConstCheck.CONST_REASSIGNED_VALUE_ERROR);
}
public void testAllChecksOn() {
CompilerOptions options = createCompilerOptions();
options.checkSuspiciousCode = true;
options.checkControlStructures = true;
options.checkRequires = CheckLevel.ERROR;
options.checkProvides = CheckLevel.ERROR;
options.generateExports = true;
options.exportTestFunctions = true;
options.closurePass = true;
options.checkMissingGetCssNameLevel = CheckLevel.ERROR;
options.checkMissingGetCssNameBlacklist = "goog";
options.syntheticBlockStartMarker = "synStart";
options.syntheticBlockEndMarker = "synEnd";
options.checkSymbols = true;
options.aggressiveVarCheck = CheckLevel.ERROR;
options.processObjectPropertyString = true;
options.collapseProperties = true;
test(options, CLOSURE_BOILERPLATE, CLOSURE_COMPILED);
}
public void testTypeCheckingWithSyntheticBlocks() {
CompilerOptions options = createCompilerOptions();
options.syntheticBlockStartMarker = "synStart";
options.syntheticBlockEndMarker = "synEnd";
options.checkTypes = true;
// We used to have a bug where the CFG drew an
// edge straight from synStart to f(progress).
// If that happens, then progress will get type {number|undefined}.
testSame(
options,
"/** @param {number} x */ function f(x) {}" +
"function g() {" +
" synStart('foo');" +
" var progress = 1;" +
" f(progress);" +
" synEnd('foo');" +
"}");
}
public void testCompilerDoesNotBlowUpIfUndefinedSymbols() {
CompilerOptions options = createCompilerOptions();
options.checkSymbols = true;
// Disable the undefined variable check.
options.setWarningLevel(
DiagnosticGroup.forType(VarCheck.UNDEFINED_VAR_ERROR),
CheckLevel.OFF);
// The compiler used to throw an IllegalStateException on this.
testSame(options, "var x = {foo: y};");
}
// Make sure that if we change variables which are constant to have
// $$constant appended to their names, we remove that tag before
// we finish.
public void testConstantTagsMustAlwaysBeRemoved() {
CompilerOptions options = createCompilerOptions();
options.variableRenaming = VariableRenamingPolicy.LOCAL;
String originalText = "var G_GEO_UNKNOWN_ADDRESS=1;\n" +
"function foo() {" +
" var localVar = 2;\n" +
" if (G_GEO_UNKNOWN_ADDRESS == localVar) {\n" +
" alert(\"A\"); }}";
String expectedText = "var G_GEO_UNKNOWN_ADDRESS=1;" +
"function foo(){var a=2;if(G_GEO_UNKNOWN_ADDRESS==a){alert(\"A\")}}";
test(options, originalText, expectedText);
}
public void testClosurePassPreservesJsDoc() {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
options.closurePass = true;
test(options,
CLOSURE_BOILERPLATE +
"goog.provide('Foo'); /** @constructor */ Foo = function() {};" +
"var x = new Foo();",
"var COMPILED=true;var goog={};goog.exportSymbol=function(){};" +
"var Foo=function(){};var x=new Foo");
test(options,
CLOSURE_BOILERPLATE +
"goog.provide('Foo'); /** @enum */ Foo = {a: 3};",
TypeCheck.ENUM_NOT_CONSTANT);
}
public void testProvidedNamespaceIsConst() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.inlineConstantVars = true;
options.collapseProperties = true;
test(options,
"var goog = {}; goog.provide('foo'); " +
"function f() { foo = {};}",
"var foo = {}; function f() { foo = {}; }",
ConstCheck.CONST_REASSIGNED_VALUE_ERROR);
}
public void testProvidedNamespaceIsConst2() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.inlineConstantVars = true;
options.collapseProperties = true;
test(options,
"var goog = {}; goog.provide('foo.bar'); " +
"function f() { foo.bar = {};}",
"var foo$bar = {};" +
"function f() { foo$bar = {}; }",
ConstCheck.CONST_REASSIGNED_VALUE_ERROR);
}
public void testProvidedNamespaceIsConst3() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.inlineConstantVars = true;
options.collapseProperties = true;
test(options,
"var goog = {}; " +
"goog.provide('foo.bar'); goog.provide('foo.bar.baz'); " +
"/** @constructor */ foo.bar = function() {};" +
"/** @constructor */ foo.bar.baz = function() {};",
"var foo$bar = function(){};" +
"var foo$bar$baz = function(){};");
}
public void testProvidedNamespaceIsConst4() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.inlineConstantVars = true;
options.collapseProperties = true;
test(options,
"var goog = {}; goog.provide('foo.Bar'); " +
"var foo = {}; foo.Bar = {};",
"var foo = {}; var foo = {}; foo.Bar = {};");
}
public void testProvidedNamespaceIsConst5() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.inlineConstantVars = true;
options.collapseProperties = true;
test(options,
"var goog = {}; goog.provide('foo.Bar'); " +
"foo = {}; foo.Bar = {};",
"var foo = {}; foo = {}; foo.Bar = {};");
}
public void testProcessDefinesAlwaysOn() {
test(createCompilerOptions(),
"/** @define {boolean} */ var HI = true; HI = false;",
"var HI = false;false;");
}
public void testProcessDefinesAdditionalReplacements() {
CompilerOptions options = createCompilerOptions();
options.setDefineToBooleanLiteral("HI", false);
test(options,
"/** @define {boolean} */ var HI = true;",
"var HI = false;");
}
public void testReplaceMessages() {
CompilerOptions options = createCompilerOptions();
String prefix = "var goog = {}; goog.getMsg = function() {};";
testSame(options, prefix + "var MSG_HI = goog.getMsg('hi');");
options.messageBundle = new EmptyMessageBundle();
test(options,
prefix + "/** @desc xyz */ var MSG_HI = goog.getMsg('hi');",
prefix + "var MSG_HI = 'hi';");
}
public void testCheckGlobalNames() {
CompilerOptions options = createCompilerOptions();
options.checkGlobalNamesLevel = CheckLevel.ERROR;
test(options, "var x = {}; var y = x.z;",
CheckGlobalNames.UNDEFINED_NAME_WARNING);
}
public void testInlineGetters() {
CompilerOptions options = createCompilerOptions();
String code =
"function Foo() {} Foo.prototype.bar = function() { return 3; };" +
"var x = new Foo(); x.bar();";
testSame(options, code);
options.inlineGetters = true;
test(options, code,
"function Foo() {} Foo.prototype.bar = function() { return 3 };" +
"var x = new Foo(); 3;");
}
public void testInlineGettersWithAmbiguate() {
CompilerOptions options = createCompilerOptions();
String code =
"/** @constructor */" +
"function Foo() {}" +
"/** @type {number} */ Foo.prototype.field;" +
"Foo.prototype.getField = function() { return this.field; };" +
"/** @constructor */" +
"function Bar() {}" +
"/** @type {string} */ Bar.prototype.field;" +
"Bar.prototype.getField = function() { return this.field; };" +
"new Foo().getField();" +
"new Bar().getField();";
testSame(options, code);
options.inlineGetters = true;
test(options, code,
"function Foo() {}" +
"Foo.prototype.field;" +
"Foo.prototype.getField = function() { return this.field; };" +
"function Bar() {}" +
"Bar.prototype.field;" +
"Bar.prototype.getField = function() { return this.field; };" +
"new Foo().field;" +
"new Bar().field;");
options.checkTypes = true;
options.ambiguateProperties = true;
// Propagating the wrong type information may cause ambiguate properties
// to generate bad code.
testSame(options, code);
}
public void testInlineVariables() {
CompilerOptions options = createCompilerOptions();
String code = "function foo() {} var x = 3; foo(x);";
testSame(options, code);
options.inlineVariables = true;
test(options, code, "(function foo() {})(3);");
options.propertyRenaming = PropertyRenamingPolicy.HEURISTIC;
test(options, code, DefaultPassConfig.CANNOT_USE_PROTOTYPE_AND_VAR);
}
public void testInlineConstants() {
CompilerOptions options = createCompilerOptions();
String code = "function foo() {} var x = 3; foo(x); var YYY = 4; foo(YYY);";
testSame(options, code);
options.inlineConstantVars = true;
test(options, code, "function foo() {} var x = 3; foo(x); foo(4);");
}
public void testMinimizeExits() {
CompilerOptions options = createCompilerOptions();
String code =
"function f() {" +
" if (window.foo) return; window.h(); " +
"}";
testSame(options, code);
options.foldConstants = true;
test(
options, code,
"function f() {" +
" window.foo || window.h(); " +
"}");
}
public void testFoldConstants() {
CompilerOptions options = createCompilerOptions();
String code = "if (true) { window.foo(); }";
testSame(options, code);
options.foldConstants = true;
test(options, code, "window.foo();");
}
public void testRemoveUnreachableCode() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { return; f(); }";
testSame(options, code);
options.removeDeadCode = true;
test(options, code, "function f() {}");
}
public void testRemoveUnusedPrototypeProperties1() {
CompilerOptions options = createCompilerOptions();
String code = "function Foo() {} " +
"Foo.prototype.bar = function() { return new Foo(); };";
testSame(options, code);
options.removeUnusedPrototypeProperties = true;
test(options, code, "function Foo() {}");
}
public void testRemoveUnusedPrototypeProperties2() {
CompilerOptions options = createCompilerOptions();
String code = "function Foo() {} " +
"Foo.prototype.bar = function() { return new Foo(); };" +
"function f(x) { x.bar(); }";
testSame(options, code);
options.removeUnusedPrototypeProperties = true;
testSame(options, code);
options.removeUnusedVars = true;
test(options, code, "");
}
public void testSmartNamePass() {
CompilerOptions options = createCompilerOptions();
String code = "function Foo() { this.bar(); } " +
"Foo.prototype.bar = function() { return Foo(); };";
testSame(options, code);
options.smartNameRemoval = true;
test(options, code, "");
}
public void testDeadAssignmentsElimination() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { var x = 3; 4; x = 5; return x; } f(); ";
testSame(options, code);
options.deadAssignmentElimination = true;
testSame(options, code);
options.removeUnusedVars = true;
test(options, code, "function f() { var x = 3; 4; x = 5; return x; } f();");
}
public void testInlineFunctions() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { return 3; } f(); ";
testSame(options, code);
options.inlineFunctions = true;
test(options, code, "3;");
}
public void testRemoveUnusedVars1() {
CompilerOptions options = createCompilerOptions();
String code = "function f(x) {} f();";
testSame(options, code);
options.removeUnusedVars = true;
test(options, code, "function f() {} f();");
}
public void testRemoveUnusedVars2() {
CompilerOptions options = createCompilerOptions();
String code = "(function f(x) {})();var g = function() {}; g();";
testSame(options, code);
options.removeUnusedVars = true;
test(options, code, "(function() {})();var g = function() {}; g();");
options.anonymousFunctionNaming = AnonymousFunctionNamingPolicy.UNMAPPED;
test(options, code, "(function f() {})();var g = function $g$() {}; g();");
}
public void testCrossModuleCodeMotion() {
CompilerOptions options = createCompilerOptions();
String[] code = new String[] {
"var x = 1;",
"x;",
};
testSame(options, code);
options.crossModuleCodeMotion = true;
test(options, code, new String[] {
"",
"var x = 1; x;",
});
}
public void testCrossModuleMethodMotion() {
CompilerOptions options = createCompilerOptions();
String[] code = new String[] {
"var Foo = function() {}; Foo.prototype.bar = function() {};" +
"var x = new Foo();",
"x.bar();",
};
testSame(options, code);
options.crossModuleMethodMotion = true;
test(options, code, new String[] {
CrossModuleMethodMotion.STUB_DECLARATIONS +
"var Foo = function() {};" +
"Foo.prototype.bar=JSCompiler_stubMethod(0); var x=new Foo;",
"Foo.prototype.bar=JSCompiler_unstubMethod(0,function(){}); x.bar()",
});
}
public void testFlowSensitiveInlineVariables1() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { var x = 3; x = 5; return x; }";
testSame(options, code);
options.flowSensitiveInlineVariables = true;
test(options, code, "function f() { var x = 3; return 5; }");
String unusedVar = "function f() { var x; x = 5; return x; } f()";
test(options, unusedVar, "function f() { var x; return 5; } f()");
options.removeUnusedVars = true;
test(options, unusedVar, "function f() { return 5; } f()");
}
public void testFlowSensitiveInlineVariables2() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.SIMPLE_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
test(options,
"function f () {\n" +
" var ab = 0;\n" +
" ab += '-';\n" +
" alert(ab);\n" +
"}",
"function f () {\n" +
" alert('0-');\n" +
"}");
}
public void testCollapseAnonymousFunctions() {
CompilerOptions options = createCompilerOptions();
String code = "var f = function() {};";
testSame(options, code);
options.collapseAnonymousFunctions = true;
test(options, code, "function f() {}");
}
public void testMoveFunctionDeclarations() {
CompilerOptions options = createCompilerOptions();
String code = "var x = f(); function f() { return 3; }";
testSame(options, code);
options.moveFunctionDeclarations = true;
test(options, code, "function f() { return 3; } var x = f();");
}
public void testNameAnonymousFunctions() {
CompilerOptions options = createCompilerOptions();
String code = "var f = function() {};";
testSame(options, code);
options.anonymousFunctionNaming = AnonymousFunctionNamingPolicy.MAPPED;
test(options, code, "var f = function $() {}");
assertNotNull(lastCompiler.getResult().namedAnonFunctionMap);
options.anonymousFunctionNaming = AnonymousFunctionNamingPolicy.UNMAPPED;
test(options, code, "var f = function $f$() {}");
assertNull(lastCompiler.getResult().namedAnonFunctionMap);
}
public void testExtractPrototypeMemberDeclarations() {
CompilerOptions options = createCompilerOptions();
String code = "var f = function() {};";
String expected = "var a; var b = function() {}; a = b.prototype;";
for (int i = 0; i < 10; i++) {
code += "f.prototype.a = " + i + ";";
expected += "a.a = " + i + ";";
}
testSame(options, code);
options.extractPrototypeMemberDeclarations = true;
options.variableRenaming = VariableRenamingPolicy.ALL;
test(options, code, expected);
options.propertyRenaming = PropertyRenamingPolicy.HEURISTIC;
options.variableRenaming = VariableRenamingPolicy.OFF;
testSame(options, code);
}
public void testDevirtualizationAndExtractPrototypeMemberDeclarations() {
CompilerOptions options = createCompilerOptions();
options.devirtualizePrototypeMethods = true;
options.collapseAnonymousFunctions = true;
options.extractPrototypeMemberDeclarations = true;
options.variableRenaming = VariableRenamingPolicy.ALL;
String code = "var f = function() {};";
String expected = "var a; function b() {} a = b.prototype;";
for (int i = 0; i < 10; i++) {
code += "f.prototype.argz = function() {arguments};";
code += "f.prototype.devir" + i + " = function() {};";
char letter = (char) ('d' + i);
expected += "a.argz = function() {arguments};";
expected += "function " + letter + "(c){}";
}
code += "var F = new f(); F.argz();";
expected += "var n = new b(); n.argz();";
for (int i = 0; i < 10; i++) {
code += "F.devir" + i + "();";
char letter = (char) ('d' + i);
expected += letter + "(n);";
}
test(options, code, expected);
}
public void testCoalesceVariableNames() {
CompilerOptions options = createCompilerOptions();
String code = "function f() {var x = 3; var y = x; var z = y; return z;}";
testSame(options, code);
options.coalesceVariableNames = true;
test(options, code,
"function f() {var x = 3; x = x; x = x; return x;}");
}
public void testPropertyRenaming() {
CompilerOptions options = createCompilerOptions();
options.propertyAffinity = true;
String code =
"function f() { return this.foo + this['bar'] + this.Baz; }" +
"f.prototype.bar = 3; f.prototype.Baz = 3;";
String heuristic =
"function f() { return this.foo + this['bar'] + this.a; }" +
"f.prototype.bar = 3; f.prototype.a = 3;";
String aggHeuristic =
"function f() { return this.foo + this['b'] + this.a; } " +
"f.prototype.b = 3; f.prototype.a = 3;";
String all =
"function f() { return this.b + this['bar'] + this.a; }" +
"f.prototype.c = 3; f.prototype.a = 3;";
testSame(options, code);
options.propertyRenaming = PropertyRenamingPolicy.HEURISTIC;
test(options, code, heuristic);
options.propertyRenaming = PropertyRenamingPolicy.AGGRESSIVE_HEURISTIC;
test(options, code, aggHeuristic);
options.propertyRenaming = PropertyRenamingPolicy.ALL_UNQUOTED;
test(options, code, all);
}
public void testConvertToDottedProperties() {
CompilerOptions options = createCompilerOptions();
String code =
"function f() { return this['bar']; } f.prototype.bar = 3;";
String expected =
"function f() { return this.bar; } f.prototype.a = 3;";
testSame(options, code);
options.convertToDottedProperties = true;
options.propertyRenaming = PropertyRenamingPolicy.ALL_UNQUOTED;
test(options, code, expected);
}
public void testRewriteFunctionExpressions() {
CompilerOptions options = createCompilerOptions();
String code = "var a = function() {};";
String expected = "function JSCompiler_emptyFn(){return function(){}} " +
"var a = JSCompiler_emptyFn();";
for (int i = 0; i < 10; i++) {
code += "a = function() {};";
expected += "a = JSCompiler_emptyFn();";
}
testSame(options, code);
options.rewriteFunctionExpressions = true;
test(options, code, expected);
}
public void testAliasAllStrings() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { return 'a'; }";
String expected = "var $$S_a = 'a'; function f() { return $$S_a; }";
testSame(options, code);
options.aliasAllStrings = true;
test(options, code, expected);
}
public void testAliasExterns() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { return window + window + window + window; }";
String expected = "var GLOBAL_window = window;" +
"function f() { return GLOBAL_window + GLOBAL_window + " +
" GLOBAL_window + GLOBAL_window; }";
testSame(options, code);
options.aliasExternals = true;
test(options, code, expected);
}
public void testAliasKeywords() {
CompilerOptions options = createCompilerOptions();
String code =
"function f() { return true + true + true + true + true + true; }";
String expected = "var JSCompiler_alias_TRUE = true;" +
"function f() { return JSCompiler_alias_TRUE + " +
" JSCompiler_alias_TRUE + JSCompiler_alias_TRUE + " +
" JSCompiler_alias_TRUE + JSCompiler_alias_TRUE + " +
" JSCompiler_alias_TRUE; }";
testSame(options, code);
options.aliasKeywords = true;
test(options, code, expected);
}
public void testRenameVars1() {
CompilerOptions options = createCompilerOptions();
String code =
"var abc = 3; function f() { var xyz = 5; return abc + xyz; }";
String local = "var abc = 3; function f() { var a = 5; return abc + a; }";
String all = "var a = 3; function c() { var b = 5; return a + b; }";
testSame(options, code);
options.variableRenaming = VariableRenamingPolicy.LOCAL;
test(options, code, local);
options.variableRenaming = VariableRenamingPolicy.ALL;
test(options, code, all);
options.reserveRawExports = true;
}
public void testRenameVars2() {
CompilerOptions options = createCompilerOptions();
options.variableRenaming = VariableRenamingPolicy.ALL;
String code = "var abc = 3; function f() { window['a'] = 5; }";
String noexport = "var a = 3; function b() { window['a'] = 5; }";
String export = "var b = 3; function c() { window['a'] = 5; }";
options.reserveRawExports = false;
test(options, code, noexport);
options.reserveRawExports = true;
test(options, code, export);
}
public void testShadowVaribles() {
CompilerOptions options = createCompilerOptions();
options.variableRenaming = VariableRenamingPolicy.LOCAL;
options.shadowVariables = true;
String code = "var f = function(x) { return function(y) {}}";
String expected = "var f = function(a) { return function(a) {}}";
test(options, code, expected);
}
public void testRenameLabels() {
CompilerOptions options = createCompilerOptions();
String code = "longLabel: while (true) { break longLabel; }";
String expected = "a: while (true) { break a; }";
testSame(options, code);
options.labelRenaming = true;
test(options, code, expected);
}
public void testBadBreakStatementInIdeMode() {
// Ensure that type-checking doesn't crash, even if the CFG is malformed.
// This can happen in IDE mode.
CompilerOptions options = createCompilerOptions();
options.ideMode = true;
options.checkTypes = true;
test(options,
"function f() { try { } catch(e) { break; } }",
RhinoErrorReporter.PARSE_ERROR);
}
public void testIssue63SourceMap() {
CompilerOptions options = createCompilerOptions();
String code = "var a;";
options.skipAllPasses = true;
options.sourceMapOutputPath = "./src.map";
Compiler compiler = compile(options, code);
compiler.toSource();
}
public void testRegExp1() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = "/(a)/.test(\"a\");";
testSame(options, code);
options.computeFunctionSideEffects = true;
String expected = "";
test(options, code, expected);
}
public void testRegExp2() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = "/(a)/.test(\"a\");var a = RegExp.$1";
testSame(options, code);
options.computeFunctionSideEffects = true;
test(options, code, CheckRegExp.REGEXP_REFERENCE);
options.setWarningLevel(DiagnosticGroups.CHECK_REGEXP, CheckLevel.OFF);
testSame(options, code);
}
public void testFoldLocals1() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
// An external object, whose constructor has no side-effects,
// and whose method "go" only modifies the object.
String code = "new Widget().go();";
testSame(options, code);
options.computeFunctionSideEffects = true;
test(options, code, "");
}
public void testFoldLocals2() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
options.checkTypes = true;
// An external function that returns a local object that the
// method "go" that only modifies the object.
String code = "widgetToken().go();";
testSame(options, code);
options.computeFunctionSideEffects = true;
test(options, code, "widgetToken()");
}
public void testFoldLocals3() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
// A function "f" who returns a known local object, and a method that
// modifies only modifies that.
String definition = "function f(){return new Widget()}";
String call = "f().go();";
String code = definition + call;
testSame(options, code);
options.computeFunctionSideEffects = true;
// BROKEN
//test(options, code, definition);
testSame(options, code);
}
public void testFoldLocals4() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = "/** @constructor */\n"
+ "function InternalWidget(){this.x = 1;}"
+ "InternalWidget.prototype.internalGo = function (){this.x = 2};"
+ "new InternalWidget().internalGo();";
testSame(options, code);
options.computeFunctionSideEffects = true;
String optimized = ""
+ "function InternalWidget(){this.x = 1;}"
+ "InternalWidget.prototype.internalGo = function (){this.x = 2};";
test(options, code, optimized);
}
public void testFoldLocals5() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = ""
+ "function fn(){var a={};a.x={};return a}"
+ "fn().x.y = 1;";
// "fn" returns a unescaped local object, we should be able to fold it,
// but we don't currently.
String result = ""
+ "function fn(){var a={x:{}};return a}"
+ "fn().x.y = 1;";
test(options, code, result);
options.computeFunctionSideEffects = true;
test(options, code, result);
}
public void testFoldLocals6() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = ""
+ "function fn(){return {}}"
+ "fn().x.y = 1;";
testSame(options, code);
options.computeFunctionSideEffects = true;
testSame(options, code);
}
public void testFoldLocals7() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = ""
+ "function InternalWidget(){return [];}"
+ "Array.prototype.internalGo = function (){this.x = 2};"
+ "InternalWidget().internalGo();";
testSame(options, code);
options.computeFunctionSideEffects = true;
String optimized = ""
+ "function InternalWidget(){return [];}"
+ "Array.prototype.internalGo = function (){this.x = 2};";
test(options, code, optimized);
}
public void testVarDeclarationsIntoFor() {
CompilerOptions options = createCompilerOptions();
options.collapseVariableDeclarations = false;
String code = "var a = 1; for (var b = 2; ;) {}";
testSame(options, code);
options.collapseVariableDeclarations = false;
test(options, code, "for (var a = 1, b = 2; ;) {}");
}
public void testExploitAssigns() {
CompilerOptions options = createCompilerOptions();
options.collapseVariableDeclarations = false;
String code = "a = 1; b = a; c = b";
testSame(options, code);
options.collapseVariableDeclarations = true;
test(options, code, "c=b=a=1");
}
public void testRecoverOnBadExterns() throws Exception {
// This test is for a bug in a very narrow set of circumstances:
// 1) externs validation has to be off.
// 2) aliasExternals has to be on.
// 3) The user has to reference a "normal" variable in externs.
// This case is handled at checking time by injecting a
// synthetic extern variable, and adding a "@suppress {duplicate}" to
// the normal code at compile time. But optimizations may remove that
// annotation, so we need to make sure that the variable declarations
// are de-duped before that happens.
CompilerOptions options = createCompilerOptions();
options.aliasExternals = true;
externs = new JSSourceFile[] {
JSSourceFile.fromCode("externs", "extern.foo")
};
test(options,
"var extern; " +
"function f() { return extern + extern + extern + extern; }",
"var extern; " +
"function f() { return extern + extern + extern + extern; }",
VarCheck.UNDEFINED_EXTERN_VAR_ERROR);
}
public void testDuplicateVariablesInExterns() {
CompilerOptions options = createCompilerOptions();
options.checkSymbols = true;
externs = new JSSourceFile[] {
JSSourceFile.fromCode("externs",
"var externs = {}; /** @suppress {duplicate} */ var externs = {};")
};
testSame(options, "");
}
public void testLanguageMode() {
CompilerOptions options = createCompilerOptions();
options.setLanguageIn(LanguageMode.ECMASCRIPT3);
String code = "var a = {get f(){}}";
Compiler compiler = compile(options, code);
checkUnexpectedErrorsOrWarnings(compiler, 1);
assertEquals(
"JSC_PARSE_ERROR. Parse error. " +
"getters are not supported in Internet Explorer " +
"at i0 line 1 : 0",
compiler.getErrors()[0].toString());
options.setLanguageIn(LanguageMode.ECMASCRIPT5);
testSame(options, code);
options.setLanguageIn(LanguageMode.ECMASCRIPT5_STRICT);
testSame(options, code);
}
public void testLanguageMode2() {
CompilerOptions options = createCompilerOptions();
options.setLanguageIn(LanguageMode.ECMASCRIPT3);
options.setWarningLevel(DiagnosticGroups.ES5_STRICT, CheckLevel.OFF);
String code = "var a = 2; delete a;";
testSame(options, code);
options.setLanguageIn(LanguageMode.ECMASCRIPT5);
testSame(options, code);
options.setLanguageIn(LanguageMode.ECMASCRIPT5_STRICT);
test(options,
code,
code,
StrictModeCheck.DELETE_VARIABLE);
}
public void testIssue598() {
CompilerOptions options = createCompilerOptions();
options.setLanguageIn(LanguageMode.ECMASCRIPT5_STRICT);
WarningLevel.VERBOSE.setOptionsForWarningLevel(options);
options.setLanguageIn(LanguageMode.ECMASCRIPT5);
String code =
"'use strict';\n" +
"function App() {}\n" +
"App.prototype = {\n" +
" get appData() { return this.appData_; },\n" +
" set appData(data) { this.appData_ = data; }\n" +
"};";
Compiler compiler = compile(options, code);
testSame(options, code);
}
public void testCoaleseVariables() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = false;
options.coalesceVariableNames = true;
String code =
"function f(a) {" +
" if (a) {" +
" return a;" +
" } else {" +
" var b = a;" +
" return b;" +
" }" +
" return a;" +
"}";
String expected =
"function f(a) {" +
" if (a) {" +
" return a;" +
" } else {" +
" a = a;" +
" return a;" +
" }" +
" return a;" +
"}";
test(options, code, expected);
options.foldConstants = true;
options.coalesceVariableNames = false;
code =
"function f(a) {" +
" if (a) {" +
" return a;" +
" } else {" +
" var b = a;" +
" return b;" +
" }" +
" return a;" +
"}";
expected =
"function f(a) {" +
" if (!a) {" +
" var b = a;" +
" return b;" +
" }" +
" return a;" +
"}";
test(options, code, expected);
options.foldConstants = true;
options.coalesceVariableNames = true;
expected =
"function f(a) {" +
" return a;" +
"}";
test(options, code, expected);
}
public void testLateStatementFusion() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
test(options,
"while(a){a();if(b){b();b()}}",
"for(;a;)a(),b&&(b(),b())");
}
public void testLateConstantReordering() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
test(options,
"if (x < 1 || x > 1 || 1 < x || 1 > x) { alert(x) }",
" (1 > x || 1 < x || 1 < x || 1 > x) && alert(x) ");
}
public void testsyntheticBlockOnDeadAssignments() {
CompilerOptions options = createCompilerOptions();
options.deadAssignmentElimination = true;
options.removeUnusedVars = true;
options.syntheticBlockStartMarker = "START";
options.syntheticBlockEndMarker = "END";
test(options, "var x; x = 1; START(); x = 1;END();x()",
"var x; x = 1;{START();{x = 1}END()}x()");
}
public void testBug4152835() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
options.syntheticBlockStartMarker = "START";
options.syntheticBlockEndMarker = "END";
test(options, "START();END()", "{START();{}END()}");
}
public void testBug5786871() {
CompilerOptions options = createCompilerOptions();
options.ideMode = true;
test(options, "function () {}", RhinoErrorReporter.PARSE_ERROR);
}
public void testIssue378() {
CompilerOptions options = createCompilerOptions();
options.inlineVariables = true;
options.flowSensitiveInlineVariables = true;
testSame(options, "function f(c) {var f = c; arguments[0] = this;" +
" f.apply(this, arguments); return this;}");
}
public void testIssue550() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.SIMPLE_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
options.foldConstants = true;
options.inlineVariables = true;
options.flowSensitiveInlineVariables = true;
test(options,
"function f(h) {\n" +
" var a = h;\n" +
" a = a + 'x';\n" +
" a = a + 'y';\n" +
" return a;\n" +
"}",
"function f(a) {return a + 'xy'}");
}
public void testIssue284() {
CompilerOptions options = createCompilerOptions();
options.smartNameRemoval = true;
test(options,
"var goog = {};" +
"goog.inherits = function(x, y) {};" +
"var ns = {};" +
"/** @constructor */" +
"ns.PageSelectionModel = function() {};" +
"/** @constructor */" +
"ns.PageSelectionModel.FooEvent = function() {};" +
"/** @constructor */" +
"ns.PageSelectionModel.SelectEvent = function() {};" +
"goog.inherits(ns.PageSelectionModel.ChangeEvent," +
" ns.PageSelectionModel.FooEvent);",
"");
}
public void testCodingConvention() {
Compiler compiler = new Compiler();
compiler.initOptions(new CompilerOptions());
assertEquals(
compiler.getCodingConvention().getClass().toString(),
ClosureCodingConvention.class.toString());
}
public void testJQueryStringSplitLoops() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
test(options,
"var x=['1','2','3','4','5','6','7']",
"var x='1,2,3,4,5,6,7'.split(',')");
options = createCompilerOptions();
options.foldConstants = true;
options.computeFunctionSideEffects = false;
options.removeUnusedVars = true;
// If we do splits too early, it would add a sideeffect to x.
test(options,
"var x=['1','2','3','4','5','6','7']",
"");
}
public void testAlwaysRunSafetyCheck() {
CompilerOptions options = createCompilerOptions();
options.checkSymbols = false;
options.customPasses = ArrayListMultimap.create();
options.customPasses.put(
CustomPassExecutionTime.BEFORE_OPTIMIZATIONS,
new CompilerPass() {
@Override public void process(Node externs, Node root) {
Node var = root.getLastChild().getFirstChild();
assertEquals(Token.VAR, var.getType());
var.detachFromParent();
}
});
try {
test(options,
"var x = 3; function f() { return x + z; }",
"function f() { return x + z; }");
fail("Expected runtime exception");
} catch (RuntimeException e) {
assertTrue(e.getMessage().indexOf("Unexpected variable x") != -1);
}
}
public void testSuppressEs5StrictWarning() {
CompilerOptions options = createCompilerOptions();
options.setWarningLevel(DiagnosticGroups.ES5_STRICT, CheckLevel.WARNING);
testSame(options,
"/** @suppress{es5Strict} */\n" +
"function f() { var arguments; }");
}
public void testCheckProvidesWarning() {
CompilerOptions options = createCompilerOptions();
options.setWarningLevel(DiagnosticGroups.CHECK_PROVIDES, CheckLevel.WARNING);
options.setCheckProvides(CheckLevel.WARNING);
test(options,
"/** @constructor */\n" +
"function f() { var arguments; }",
DiagnosticType.warning("JSC_MISSING_PROVIDE", "missing goog.provide(''{0}'')"));
}
public void testSuppressCheckProvidesWarning() {
CompilerOptions options = createCompilerOptions();
options.setWarningLevel(DiagnosticGroups.CHECK_PROVIDES, CheckLevel.WARNING);
options.setCheckProvides(CheckLevel.WARNING);
testSame(options,
"/** @constructor\n" +
" * @suppress{checkProvides} */\n" +
"function f() { var arguments; }");
}
public void testRenamePrefixNamespace() {
String code =
"var x = {}; x.FOO = 5; x.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.collapseProperties = true;
options.renamePrefixNamespace = "_";
test(options, code, "_.x$FOO = 5; _.x$bar = 3;");
}
public void testRenamePrefixNamespaceActivatesMoveFunctionDeclarations() {
CompilerOptions options = createCompilerOptions();
String code = "var x = f; function f() { return 3; }";
testSame(options, code);
assertFalse(options.moveFunctionDeclarations);
options.renamePrefixNamespace = "_";
test(options, code, "_.f = function() { return 3; }; _.x = _.f;");
}
public void testBrokenNameSpace() {
CompilerOptions options = createCompilerOptions();
String code = "var goog; goog.provide('i.am.on.a.Horse');" +
"i.am.on.a.Horse = function() {};" +
"i.am.on.a.Horse.prototype.x = function() {};" +
"i.am.on.a.Boat.prototype.y = function() {}";
options.closurePass = true;
options.collapseProperties = true;
options.smartNameRemoval = true;
test(options, code, "");
}
public void testNamelessParameter() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.ADVANCED_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
String code =
"var impl_0;" +
"$load($init());" +
"function $load(){" +
" window['f'] = impl_0;" +
"}" +
"function $init() {" +
" impl_0 = {};" +
"}";
String result =
"window.f = {};";
test(options, code, result);
}
public void testHiddenSideEffect() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.ADVANCED_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
options.setAliasExternals(true);
String code =
"window.offsetWidth;";
String result =
"window.offsetWidth;";
test(options, code, result);
}
public void testNegativeZero() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.ADVANCED_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
test(options,
"function bar(x) { return x; }\n" +
"function foo(x) { print(x / bar(0));\n" +
" print(x / bar(-0)); }\n" +
"foo(3);",
"print(3/0);print(3/-0);");
}
private void testSame(CompilerOptions options, String original) {
testSame(options, new String[] { original });
}
private void testSame(CompilerOptions options, String[] original) {
test(options, original, original);
}
/**
* Asserts that when compiling with the given compiler options,
* {@code original} is transformed into {@code compiled}.
*/
private void test(CompilerOptions options,
String original, String compiled) {
test(options, new String[] { original }, new String[] { compiled });
}
/**
* Asserts that when compiling with the given compiler options,
* {@code original} is transformed into {@code compiled}.
*/
private void test(CompilerOptions options,
String[] original, String[] compiled) {
Compiler compiler = compile(options, original);
assertEquals("Expected no warnings or errors\n" +
"Errors: \n" + Joiner.on("\n").join(compiler.getErrors()) +
"Warnings: \n" + Joiner.on("\n").join(compiler.getWarnings()),
0, compiler.getErrors().length + compiler.getWarnings().length);
Node root = compiler.getRoot().getLastChild();
Node expectedRoot = parse(compiled, options);
String explanation = expectedRoot.checkTreeEquals(root);
assertNull("\nExpected: " + compiler.toSource(expectedRoot) +
"\nResult: " + compiler.toSource(root) +
"\n" + explanation, explanation);
}
/**
* Asserts that when compiling with the given compiler options,
* there is an error or warning.
*/
private void test(CompilerOptions options,
String original, DiagnosticType warning) {
test(options, new String[] { original }, warning);
}
private void test(CompilerOptions options,
String original, String compiled, DiagnosticType warning) {
test(options, new String[] { original }, new String[] { compiled },
warning);
}
private void test(CompilerOptions options,
String[] original, DiagnosticType warning) {
test(options, original, null, warning);
}
/**
* Asserts that when compiling with the given compiler options,
* there is an error or warning.
*/
private void test(CompilerOptions options,
String[] original, String[] compiled, DiagnosticType warning) {
Compiler compiler = compile(options, original);
checkUnexpectedErrorsOrWarnings(compiler, 1);
assertEquals("Expected exactly one warning or error",
1, compiler.getErrors().length + compiler.getWarnings().length);
if (compiler.getErrors().length > 0) {
assertEquals(warning, compiler.getErrors()[0].getType());
} else {
assertEquals(warning, compiler.getWarnings()[0].getType());
}
if (compiled != null) {
Node root = compiler.getRoot().getLastChild();
Node expectedRoot = parse(compiled, options);
String explanation = expectedRoot.checkTreeEquals(root);
assertNull("\nExpected: " + compiler.toSource(expectedRoot) +
"\nResult: " + compiler.toSource(root) +
"\n" + explanation, explanation);
}
}
private void checkUnexpectedErrorsOrWarnings(
Compiler compiler, int expected) {
int actual = compiler.getErrors().length + compiler.getWarnings().length;
if (actual != expected) {
String msg = "";
for (JSError err : compiler.getErrors()) {
msg += "Error:" + err.toString() + "\n";
}
for (JSError err : compiler.getWarnings()) {
msg += "Warning:" + err.toString() + "\n";
}
assertEquals("Unexpected warnings or errors.\n "+ msg,
expected, actual);
}
}
private Compiler compile(CompilerOptions options, String original) {
return compile(options, new String[] { original });
}
private Compiler compile(CompilerOptions options, String[] original) {
Compiler compiler = lastCompiler = new Compiler();
JSSourceFile[] inputs = new JSSourceFile[original.length];
for (int i = 0; i < original.length; i++) {
inputs[i] = JSSourceFile.fromCode("input" + i, original[i]);
}
compiler.compile(
externs, CompilerTestCase.createModuleChain(original), options);
return compiler;
}
private Node parse(String[] original, CompilerOptions options) {
Compiler compiler = new Compiler();
JSSourceFile[] inputs = new JSSourceFile[original.length];
for (int i = 0; i < inputs.length; i++) {
inputs[i] = JSSourceFile.fromCode("input" + i, original[i]);
}
compiler.init(externs, inputs, options);
checkUnexpectedErrorsOrWarnings(compiler, 0);
Node all = compiler.parseInputs();
checkUnexpectedErrorsOrWarnings(compiler, 0);
Node n = all.getLastChild();
Node externs = all.getFirstChild();
(new CreateSyntheticBlocks(
compiler, "synStart", "synEnd")).process(externs, n);
(new Normalize(compiler, false)).process(externs, n);
(MakeDeclaredNamesUnique.getContextualRenameInverter(compiler)).process(
externs, n);
(new Denormalize(compiler)).process(externs, n);
return n;
}
/** Creates a CompilerOptions object with google coding conventions. */
private CompilerOptions createCompilerOptions() {
CompilerOptions options = new CompilerOptions();
options.setCodingConvention(new GoogleCodingConvention());
return options;
}
} | // You are a professional Java test case writer, please create a test case named `testIssue284` for the issue `Closure-284`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-284
//
// ## Issue-Title:
// smartNameRemoval causing compiler crash
//
// ## Issue-Description:
// **What steps will reproduce the problem?**
// Compiler the following code in advanced mode:
//
// {{{
// var goog = {};
// goog.inherits = function(x, y) {};
// var ns = {};
// /\*\* @constructor \*/ ns.PageSelectionModel = function(){};
//
// /\*\* @constructor \*/
// ns.PageSelectionModel.FooEvent = function() {};
// /\*\* @constructor \*/
// ns.PageSelectionModel.SelectEvent = function() {};
// goog.inherits(ns.PageSelectionModel.ChangeEvent, ns.PageSelectionModel.FooEvent);
// }}}
//
//
// **What is the expected output? What do you see instead?**
// The compiler will crash. The last var check throws an illegal state exception because it knows something is wrong.
//
// The crash is caused by smartNameRemoval. It has special logic for counting references in class-defining function calls (like goog.inherits), and it isn't properly creating a reference to PageSelectionModel.
//
//
public void testIssue284() {
| 1,781 | 40 | 1,765 | test/com/google/javascript/jscomp/IntegrationTest.java | test | ```markdown
## Issue-ID: Closure-284
## Issue-Title:
smartNameRemoval causing compiler crash
## Issue-Description:
**What steps will reproduce the problem?**
Compiler the following code in advanced mode:
{{{
var goog = {};
goog.inherits = function(x, y) {};
var ns = {};
/\*\* @constructor \*/ ns.PageSelectionModel = function(){};
/\*\* @constructor \*/
ns.PageSelectionModel.FooEvent = function() {};
/\*\* @constructor \*/
ns.PageSelectionModel.SelectEvent = function() {};
goog.inherits(ns.PageSelectionModel.ChangeEvent, ns.PageSelectionModel.FooEvent);
}}}
**What is the expected output? What do you see instead?**
The compiler will crash. The last var check throws an illegal state exception because it knows something is wrong.
The crash is caused by smartNameRemoval. It has special logic for counting references in class-defining function calls (like goog.inherits), and it isn't properly creating a reference to PageSelectionModel.
```
You are a professional Java test case writer, please create a test case named `testIssue284` for the issue `Closure-284`, utilizing the provided issue report information and the following function signature.
```java
public void testIssue284() {
```
| 1,765 | [
"com.google.javascript.jscomp.NameAnalyzer"
] | 7ed74e2a02ac5a5c5d87c50e8d177187d5208dbf8fb09783a33416c19abf0b5a | public void testIssue284() | // You are a professional Java test case writer, please create a test case named `testIssue284` for the issue `Closure-284`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-284
//
// ## Issue-Title:
// smartNameRemoval causing compiler crash
//
// ## Issue-Description:
// **What steps will reproduce the problem?**
// Compiler the following code in advanced mode:
//
// {{{
// var goog = {};
// goog.inherits = function(x, y) {};
// var ns = {};
// /\*\* @constructor \*/ ns.PageSelectionModel = function(){};
//
// /\*\* @constructor \*/
// ns.PageSelectionModel.FooEvent = function() {};
// /\*\* @constructor \*/
// ns.PageSelectionModel.SelectEvent = function() {};
// goog.inherits(ns.PageSelectionModel.ChangeEvent, ns.PageSelectionModel.FooEvent);
// }}}
//
//
// **What is the expected output? What do you see instead?**
// The compiler will crash. The last var check throws an illegal state exception because it knows something is wrong.
//
// The crash is caused by smartNameRemoval. It has special logic for counting references in class-defining function calls (like goog.inherits), and it isn't properly creating a reference to PageSelectionModel.
//
//
| Closure | /*
* Copyright 2009 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import com.google.common.base.Joiner;
import com.google.common.collect.ArrayListMultimap;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.Sets;
import com.google.javascript.jscomp.CompilerOptions.LanguageMode;
import com.google.javascript.rhino.Node;
import com.google.javascript.rhino.Token;
import junit.framework.TestCase;
/**
* Tests for {@link PassFactory}.
*
* @author [email protected] (Nick Santos)
*/
public class IntegrationTest extends TestCase {
/** Externs for the test */
private final JSSourceFile[] DEFAULT_EXTERNS = new JSSourceFile[] {
JSSourceFile.fromCode("externs",
"var arguments;\n"
+ "/** @constructor */ function Window() {}\n"
+ "/** @type {string} */ Window.prototype.name;\n"
+ "/** @type {string} */ Window.prototype.offsetWidth;\n"
+ "/** @type {Window} */ var window;\n"
+ "/** @nosideeffects */ function noSideEffects() {}\n"
+ "/** @constructor\n * @nosideeffects */ function Widget() {}\n"
+ "/** @modifies {this} */ Widget.prototype.go = function() {};\n"
+ "/** @return {string} */ var widgetToken = function() {};\n")
};
private JSSourceFile[] externs = DEFAULT_EXTERNS;
private static final String CLOSURE_BOILERPLATE =
"/** @define {boolean} */ var COMPILED = false; var goog = {};" +
"goog.exportSymbol = function() {};";
private static final String CLOSURE_COMPILED =
"var COMPILED = true; var goog$exportSymbol = function() {};";
// The most recently used compiler.
private Compiler lastCompiler;
@Override
public void setUp() {
externs = DEFAULT_EXTERNS;
lastCompiler = null;
}
public void testBug1949424() {
CompilerOptions options = createCompilerOptions();
options.collapseProperties = true;
options.closurePass = true;
test(options, CLOSURE_BOILERPLATE + "goog.provide('FOO'); FOO.bar = 3;",
CLOSURE_COMPILED + "var FOO$bar = 3;");
}
public void testBug1949424_v2() {
CompilerOptions options = createCompilerOptions();
options.collapseProperties = true;
options.closurePass = true;
test(options, CLOSURE_BOILERPLATE + "goog.provide('FOO.BAR'); FOO.BAR = 3;",
CLOSURE_COMPILED + "var FOO$BAR = 3;");
}
public void testBug1956277() {
CompilerOptions options = createCompilerOptions();
options.collapseProperties = true;
options.inlineVariables = true;
test(options, "var CONST = {}; CONST.bar = null;" +
"function f(url) { CONST.bar = url; }",
"var CONST$bar = null; function f(url) { CONST$bar = url; }");
}
public void testBug1962380() {
CompilerOptions options = createCompilerOptions();
options.collapseProperties = true;
options.inlineVariables = true;
options.generateExports = true;
test(options,
CLOSURE_BOILERPLATE + "/** @export */ goog.CONSTANT = 1;" +
"var x = goog.CONSTANT;",
"(function() {})('goog.CONSTANT', 1);" +
"var x = 1;");
}
public void testBug2410122() {
CompilerOptions options = createCompilerOptions();
options.generateExports = true;
options.closurePass = true;
test(options,
"var goog = {};" +
"function F() {}" +
"/** @export */ function G() { goog.base(this); } " +
"goog.inherits(G, F);",
"var goog = {};" +
"function F() {}" +
"function G() { F.call(this); } " +
"goog.inherits(G, F); goog.exportSymbol('G', G);");
}
public void testIssue90() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
options.inlineVariables = true;
options.removeDeadCode = true;
test(options,
"var x; x && alert(1);",
"");
}
public void testClosurePassOff() {
CompilerOptions options = createCompilerOptions();
options.closurePass = false;
testSame(
options,
"var goog = {}; goog.require = function(x) {}; goog.require('foo');");
testSame(
options,
"var goog = {}; goog.getCssName = function(x) {};" +
"goog.getCssName('foo');");
}
public void testClosurePassOn() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
test(
options,
"var goog = {}; goog.require = function(x) {}; goog.require('foo');",
ProcessClosurePrimitives.MISSING_PROVIDE_ERROR);
test(
options,
"/** @define {boolean} */ var COMPILED = false;" +
"var goog = {}; goog.getCssName = function(x) {};" +
"goog.getCssName('foo');",
"var COMPILED = true;" +
"var goog = {}; goog.getCssName = function(x) {};" +
"'foo';");
}
public void testCssNameCheck() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.checkMissingGetCssNameLevel = CheckLevel.ERROR;
options.checkMissingGetCssNameBlacklist = "foo";
test(options, "var x = 'foo';",
CheckMissingGetCssName.MISSING_GETCSSNAME);
}
public void testBug2592659() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.checkTypes = true;
options.checkMissingGetCssNameLevel = CheckLevel.WARNING;
options.checkMissingGetCssNameBlacklist = "foo";
test(options,
"var goog = {};\n" +
"/**\n" +
" * @param {string} className\n" +
" * @param {string=} opt_modifier\n" +
" * @return {string}\n" +
"*/\n" +
"goog.getCssName = function(className, opt_modifier) {}\n" +
"var x = goog.getCssName(123, 'a');",
TypeValidator.TYPE_MISMATCH_WARNING);
}
public void testTypedefBeforeOwner1() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
test(options,
"goog.provide('foo.Bar.Type');\n" +
"goog.provide('foo.Bar');\n" +
"/** @typedef {number} */ foo.Bar.Type;\n" +
"foo.Bar = function() {};",
"var foo = {}; foo.Bar.Type; foo.Bar = function() {};");
}
public void testTypedefBeforeOwner2() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.collapseProperties = true;
test(options,
"goog.provide('foo.Bar.Type');\n" +
"goog.provide('foo.Bar');\n" +
"/** @typedef {number} */ foo.Bar.Type;\n" +
"foo.Bar = function() {};",
"var foo$Bar$Type; var foo$Bar = function() {};");
}
public void testExportedNames() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.variableRenaming = VariableRenamingPolicy.ALL;
test(options,
"/** @define {boolean} */ var COMPILED = false;" +
"var goog = {}; goog.exportSymbol('b', goog);",
"var a = true; var c = {}; c.exportSymbol('b', c);");
test(options,
"/** @define {boolean} */ var COMPILED = false;" +
"var goog = {}; goog.exportSymbol('a', goog);",
"var b = true; var c = {}; c.exportSymbol('a', c);");
}
public void testCheckGlobalThisOn() {
CompilerOptions options = createCompilerOptions();
options.checkSuspiciousCode = true;
options.checkGlobalThisLevel = CheckLevel.ERROR;
test(options, "function f() { this.y = 3; }", CheckGlobalThis.GLOBAL_THIS);
}
public void testSusiciousCodeOff() {
CompilerOptions options = createCompilerOptions();
options.checkSuspiciousCode = false;
options.checkGlobalThisLevel = CheckLevel.ERROR;
test(options, "function f() { this.y = 3; }", CheckGlobalThis.GLOBAL_THIS);
}
public void testCheckGlobalThisOff() {
CompilerOptions options = createCompilerOptions();
options.checkSuspiciousCode = true;
options.checkGlobalThisLevel = CheckLevel.OFF;
testSame(options, "function f() { this.y = 3; }");
}
public void testCheckRequiresAndCheckProvidesOff() {
testSame(createCompilerOptions(), new String[] {
"/** @constructor */ function Foo() {}",
"new Foo();"
});
}
public void testCheckRequiresOn() {
CompilerOptions options = createCompilerOptions();
options.checkRequires = CheckLevel.ERROR;
test(options, new String[] {
"/** @constructor */ function Foo() {}",
"new Foo();"
}, CheckRequiresForConstructors.MISSING_REQUIRE_WARNING);
}
public void testCheckProvidesOn() {
CompilerOptions options = createCompilerOptions();
options.checkProvides = CheckLevel.ERROR;
test(options, new String[] {
"/** @constructor */ function Foo() {}",
"new Foo();"
}, CheckProvides.MISSING_PROVIDE_WARNING);
}
public void testGenerateExportsOff() {
testSame(createCompilerOptions(), "/** @export */ function f() {}");
}
public void testGenerateExportsOn() {
CompilerOptions options = createCompilerOptions();
options.generateExports = true;
test(options, "/** @export */ function f() {}",
"/** @export */ function f() {} goog.exportSymbol('f', f);");
}
public void testExportTestFunctionsOff() {
testSame(createCompilerOptions(), "function testFoo() {}");
}
public void testExportTestFunctionsOn() {
CompilerOptions options = createCompilerOptions();
options.exportTestFunctions = true;
test(options, "function testFoo() {}",
"/** @export */ function testFoo() {}" +
"goog.exportSymbol('testFoo', testFoo);");
}
public void testCheckSymbolsOff() {
CompilerOptions options = createCompilerOptions();
testSame(options, "x = 3;");
}
public void testCheckSymbolsOn() {
CompilerOptions options = createCompilerOptions();
options.checkSymbols = true;
test(options, "x = 3;", VarCheck.UNDEFINED_VAR_ERROR);
}
public void testCheckReferencesOff() {
CompilerOptions options = createCompilerOptions();
testSame(options, "x = 3; var x = 5;");
}
public void testCheckReferencesOn() {
CompilerOptions options = createCompilerOptions();
options.aggressiveVarCheck = CheckLevel.ERROR;
test(options, "x = 3; var x = 5;",
VariableReferenceCheck.UNDECLARED_REFERENCE);
}
public void testInferTypes() {
CompilerOptions options = createCompilerOptions();
options.inferTypes = true;
options.checkTypes = false;
options.closurePass = true;
test(options,
CLOSURE_BOILERPLATE +
"goog.provide('Foo'); /** @enum */ Foo = {a: 3};",
TypeCheck.ENUM_NOT_CONSTANT);
assertTrue(lastCompiler.getErrorManager().getTypedPercent() == 0);
// This does not generate a warning.
test(options, "/** @type {number} */ var n = window.name;",
"var n = window.name;");
assertTrue(lastCompiler.getErrorManager().getTypedPercent() == 0);
}
public void testTypeCheckAndInference() {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
test(options, "/** @type {number} */ var n = window.name;",
TypeValidator.TYPE_MISMATCH_WARNING);
assertTrue(lastCompiler.getErrorManager().getTypedPercent() > 0);
}
public void testTypeNameParser() {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
test(options, "/** @type {n} */ var n = window.name;",
RhinoErrorReporter.TYPE_PARSE_ERROR);
}
// This tests that the TypedScopeCreator is memoized so that it only creates a
// Scope object once for each scope. If, when type inference requests a scope,
// it creates a new one, then multiple JSType objects end up getting created
// for the same local type, and ambiguate will rename the last statement to
// o.a(o.a, o.a), which is bad.
public void testMemoizedTypedScopeCreator() {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
options.ambiguateProperties = true;
options.propertyRenaming = PropertyRenamingPolicy.ALL_UNQUOTED;
test(options, "function someTest() {\n"
+ " /** @constructor */\n"
+ " function Foo() { this.instProp = 3; }\n"
+ " Foo.prototype.protoProp = function(a, b) {};\n"
+ " /** @constructor\n @extends Foo */\n"
+ " function Bar() {}\n"
+ " goog.inherits(Bar, Foo);\n"
+ " var o = new Bar();\n"
+ " o.protoProp(o.protoProp, o.instProp);\n"
+ "}",
"function someTest() {\n"
+ " function Foo() { this.b = 3; }\n"
+ " Foo.prototype.a = function(a, b) {};\n"
+ " function Bar() {}\n"
+ " goog.c(Bar, Foo);\n"
+ " var o = new Bar();\n"
+ " o.a(o.a, o.b);\n"
+ "}");
}
public void testCheckTypes() {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
test(options, "var x = x || {}; x.f = function() {}; x.f(3);",
TypeCheck.WRONG_ARGUMENT_COUNT);
}
public void testReplaceCssNames() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.gatherCssNames = true;
test(options, "/** @define {boolean} */\n"
+ "var COMPILED = false;\n"
+ "goog.setCssNameMapping({'foo':'bar'});\n"
+ "function getCss() {\n"
+ " return goog.getCssName('foo');\n"
+ "}",
"var COMPILED = true;\n"
+ "function getCss() {\n"
+ " return \"bar\";"
+ "}");
assertEquals(
ImmutableMap.of("foo", new Integer(1)),
lastCompiler.getPassConfig().getIntermediateState().cssNames);
}
public void testRemoveClosureAsserts() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
testSame(options,
"var goog = {};"
+ "goog.asserts.assert(goog);");
options.removeClosureAsserts = true;
test(options,
"var goog = {};"
+ "goog.asserts.assert(goog);",
"var goog = {};");
}
public void testDeprecation() {
String code = "/** @deprecated */ function f() { } function g() { f(); }";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.setWarningLevel(DiagnosticGroups.DEPRECATED, CheckLevel.ERROR);
testSame(options, code);
options.checkTypes = true;
test(options, code, CheckAccessControls.DEPRECATED_NAME);
}
public void testVisibility() {
String[] code = {
"/** @private */ function f() { }",
"function g() { f(); }"
};
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.setWarningLevel(DiagnosticGroups.VISIBILITY, CheckLevel.ERROR);
testSame(options, code);
options.checkTypes = true;
test(options, code, CheckAccessControls.BAD_PRIVATE_GLOBAL_ACCESS);
}
public void testUnreachableCode() {
String code = "function f() { return \n 3; }";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.checkUnreachableCode = CheckLevel.ERROR;
test(options, code, CheckUnreachableCode.UNREACHABLE_CODE);
}
public void testMissingReturn() {
String code =
"/** @return {number} */ function f() { if (f) { return 3; } }";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.checkMissingReturn = CheckLevel.ERROR;
testSame(options, code);
options.checkTypes = true;
test(options, code, CheckMissingReturn.MISSING_RETURN_STATEMENT);
}
public void testIdGenerators() {
String code = "function f() {} f('id');";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.idGenerators = Sets.newHashSet("f");
test(options, code, "function f() {} 'a';");
}
public void testOptimizeArgumentsArray() {
String code = "function f() { return arguments[0]; }";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.optimizeArgumentsArray = true;
String argName = "JSCompiler_OptimizeArgumentsArray_p0";
test(options, code,
"function f(" + argName + ") { return " + argName + "; }");
}
public void testOptimizeParameters() {
String code = "function f(a) { return a; } f(true);";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.optimizeParameters = true;
test(options, code, "function f() { var a = true; return a;} f();");
}
public void testOptimizeReturns() {
String code = "function f(a) { return a; } f(true);";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.optimizeReturns = true;
test(options, code, "function f(a) {return;} f(true);");
}
public void testRemoveAbstractMethods() {
String code = CLOSURE_BOILERPLATE +
"var x = {}; x.foo = goog.abstractMethod; x.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.closurePass = true;
options.collapseProperties = true;
test(options, code, CLOSURE_COMPILED + " var x$bar = 3;");
}
public void testCollapseProperties1() {
String code =
"var x = {}; x.FOO = 5; x.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.collapseProperties = true;
test(options, code, "var x$FOO = 5; var x$bar = 3;");
}
public void testCollapseProperties2() {
String code =
"var x = {}; x.FOO = 5; x.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.collapseProperties = true;
options.collapseObjectLiterals = true;
test(options, code, "var x$FOO = 5; var x$bar = 3;");
}
public void testCollapseObjectLiteral1() {
// Verify collapseObjectLiterals does nothing in global scope
String code = "var x = {}; x.FOO = 5; x.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.collapseObjectLiterals = true;
testSame(options, code);
}
public void testCollapseObjectLiteral2() {
String code =
"function f() {var x = {}; x.FOO = 5; x.bar = 3;}";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.collapseObjectLiterals = true;
test(options, code,
"function f(){" +
"var JSCompiler_object_inline_FOO_0;" +
"var JSCompiler_object_inline_bar_1;" +
"JSCompiler_object_inline_FOO_0=5;" +
"JSCompiler_object_inline_bar_1=3}");
}
public void testTightenTypesWithoutTypeCheck() {
CompilerOptions options = createCompilerOptions();
options.tightenTypes = true;
test(options, "", DefaultPassConfig.TIGHTEN_TYPES_WITHOUT_TYPE_CHECK);
}
public void testDisambiguateProperties() {
String code =
"/** @constructor */ function Foo(){} Foo.prototype.bar = 3;" +
"/** @constructor */ function Baz(){} Baz.prototype.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.disambiguateProperties = true;
options.checkTypes = true;
test(options, code,
"function Foo(){} Foo.prototype.Foo_prototype$bar = 3;" +
"function Baz(){} Baz.prototype.Baz_prototype$bar = 3;");
}
public void testMarkPureCalls() {
String testCode = "function foo() {} foo();";
CompilerOptions options = createCompilerOptions();
options.removeDeadCode = true;
testSame(options, testCode);
options.computeFunctionSideEffects = true;
test(options, testCode, "function foo() {}");
}
public void testMarkNoSideEffects() {
String testCode = "noSideEffects();";
CompilerOptions options = createCompilerOptions();
options.removeDeadCode = true;
testSame(options, testCode);
options.markNoSideEffectCalls = true;
test(options, testCode, "");
}
public void testChainedCalls() {
CompilerOptions options = createCompilerOptions();
options.chainCalls = true;
test(
options,
"/** @constructor */ function Foo() {} " +
"Foo.prototype.bar = function() { return this; }; " +
"var f = new Foo();" +
"f.bar(); " +
"f.bar(); ",
"function Foo() {} " +
"Foo.prototype.bar = function() { return this; }; " +
"var f = new Foo();" +
"f.bar().bar();");
}
public void testExtraAnnotationNames() {
CompilerOptions options = createCompilerOptions();
options.setExtraAnnotationNames(Sets.newHashSet("TagA", "TagB"));
test(
options,
"/** @TagA */ var f = new Foo(); /** @TagB */ f.bar();",
"var f = new Foo(); f.bar();");
}
public void testDevirtualizePrototypeMethods() {
CompilerOptions options = createCompilerOptions();
options.devirtualizePrototypeMethods = true;
test(
options,
"/** @constructor */ var Foo = function() {}; " +
"Foo.prototype.bar = function() {};" +
"(new Foo()).bar();",
"var Foo = function() {};" +
"var JSCompiler_StaticMethods_bar = " +
" function(JSCompiler_StaticMethods_bar$self) {};" +
"JSCompiler_StaticMethods_bar(new Foo());");
}
public void testCheckConsts() {
CompilerOptions options = createCompilerOptions();
options.inlineConstantVars = true;
test(options, "var FOO = true; FOO = false",
ConstCheck.CONST_REASSIGNED_VALUE_ERROR);
}
public void testAllChecksOn() {
CompilerOptions options = createCompilerOptions();
options.checkSuspiciousCode = true;
options.checkControlStructures = true;
options.checkRequires = CheckLevel.ERROR;
options.checkProvides = CheckLevel.ERROR;
options.generateExports = true;
options.exportTestFunctions = true;
options.closurePass = true;
options.checkMissingGetCssNameLevel = CheckLevel.ERROR;
options.checkMissingGetCssNameBlacklist = "goog";
options.syntheticBlockStartMarker = "synStart";
options.syntheticBlockEndMarker = "synEnd";
options.checkSymbols = true;
options.aggressiveVarCheck = CheckLevel.ERROR;
options.processObjectPropertyString = true;
options.collapseProperties = true;
test(options, CLOSURE_BOILERPLATE, CLOSURE_COMPILED);
}
public void testTypeCheckingWithSyntheticBlocks() {
CompilerOptions options = createCompilerOptions();
options.syntheticBlockStartMarker = "synStart";
options.syntheticBlockEndMarker = "synEnd";
options.checkTypes = true;
// We used to have a bug where the CFG drew an
// edge straight from synStart to f(progress).
// If that happens, then progress will get type {number|undefined}.
testSame(
options,
"/** @param {number} x */ function f(x) {}" +
"function g() {" +
" synStart('foo');" +
" var progress = 1;" +
" f(progress);" +
" synEnd('foo');" +
"}");
}
public void testCompilerDoesNotBlowUpIfUndefinedSymbols() {
CompilerOptions options = createCompilerOptions();
options.checkSymbols = true;
// Disable the undefined variable check.
options.setWarningLevel(
DiagnosticGroup.forType(VarCheck.UNDEFINED_VAR_ERROR),
CheckLevel.OFF);
// The compiler used to throw an IllegalStateException on this.
testSame(options, "var x = {foo: y};");
}
// Make sure that if we change variables which are constant to have
// $$constant appended to their names, we remove that tag before
// we finish.
public void testConstantTagsMustAlwaysBeRemoved() {
CompilerOptions options = createCompilerOptions();
options.variableRenaming = VariableRenamingPolicy.LOCAL;
String originalText = "var G_GEO_UNKNOWN_ADDRESS=1;\n" +
"function foo() {" +
" var localVar = 2;\n" +
" if (G_GEO_UNKNOWN_ADDRESS == localVar) {\n" +
" alert(\"A\"); }}";
String expectedText = "var G_GEO_UNKNOWN_ADDRESS=1;" +
"function foo(){var a=2;if(G_GEO_UNKNOWN_ADDRESS==a){alert(\"A\")}}";
test(options, originalText, expectedText);
}
public void testClosurePassPreservesJsDoc() {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
options.closurePass = true;
test(options,
CLOSURE_BOILERPLATE +
"goog.provide('Foo'); /** @constructor */ Foo = function() {};" +
"var x = new Foo();",
"var COMPILED=true;var goog={};goog.exportSymbol=function(){};" +
"var Foo=function(){};var x=new Foo");
test(options,
CLOSURE_BOILERPLATE +
"goog.provide('Foo'); /** @enum */ Foo = {a: 3};",
TypeCheck.ENUM_NOT_CONSTANT);
}
public void testProvidedNamespaceIsConst() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.inlineConstantVars = true;
options.collapseProperties = true;
test(options,
"var goog = {}; goog.provide('foo'); " +
"function f() { foo = {};}",
"var foo = {}; function f() { foo = {}; }",
ConstCheck.CONST_REASSIGNED_VALUE_ERROR);
}
public void testProvidedNamespaceIsConst2() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.inlineConstantVars = true;
options.collapseProperties = true;
test(options,
"var goog = {}; goog.provide('foo.bar'); " +
"function f() { foo.bar = {};}",
"var foo$bar = {};" +
"function f() { foo$bar = {}; }",
ConstCheck.CONST_REASSIGNED_VALUE_ERROR);
}
public void testProvidedNamespaceIsConst3() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.inlineConstantVars = true;
options.collapseProperties = true;
test(options,
"var goog = {}; " +
"goog.provide('foo.bar'); goog.provide('foo.bar.baz'); " +
"/** @constructor */ foo.bar = function() {};" +
"/** @constructor */ foo.bar.baz = function() {};",
"var foo$bar = function(){};" +
"var foo$bar$baz = function(){};");
}
public void testProvidedNamespaceIsConst4() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.inlineConstantVars = true;
options.collapseProperties = true;
test(options,
"var goog = {}; goog.provide('foo.Bar'); " +
"var foo = {}; foo.Bar = {};",
"var foo = {}; var foo = {}; foo.Bar = {};");
}
public void testProvidedNamespaceIsConst5() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.inlineConstantVars = true;
options.collapseProperties = true;
test(options,
"var goog = {}; goog.provide('foo.Bar'); " +
"foo = {}; foo.Bar = {};",
"var foo = {}; foo = {}; foo.Bar = {};");
}
public void testProcessDefinesAlwaysOn() {
test(createCompilerOptions(),
"/** @define {boolean} */ var HI = true; HI = false;",
"var HI = false;false;");
}
public void testProcessDefinesAdditionalReplacements() {
CompilerOptions options = createCompilerOptions();
options.setDefineToBooleanLiteral("HI", false);
test(options,
"/** @define {boolean} */ var HI = true;",
"var HI = false;");
}
public void testReplaceMessages() {
CompilerOptions options = createCompilerOptions();
String prefix = "var goog = {}; goog.getMsg = function() {};";
testSame(options, prefix + "var MSG_HI = goog.getMsg('hi');");
options.messageBundle = new EmptyMessageBundle();
test(options,
prefix + "/** @desc xyz */ var MSG_HI = goog.getMsg('hi');",
prefix + "var MSG_HI = 'hi';");
}
public void testCheckGlobalNames() {
CompilerOptions options = createCompilerOptions();
options.checkGlobalNamesLevel = CheckLevel.ERROR;
test(options, "var x = {}; var y = x.z;",
CheckGlobalNames.UNDEFINED_NAME_WARNING);
}
public void testInlineGetters() {
CompilerOptions options = createCompilerOptions();
String code =
"function Foo() {} Foo.prototype.bar = function() { return 3; };" +
"var x = new Foo(); x.bar();";
testSame(options, code);
options.inlineGetters = true;
test(options, code,
"function Foo() {} Foo.prototype.bar = function() { return 3 };" +
"var x = new Foo(); 3;");
}
public void testInlineGettersWithAmbiguate() {
CompilerOptions options = createCompilerOptions();
String code =
"/** @constructor */" +
"function Foo() {}" +
"/** @type {number} */ Foo.prototype.field;" +
"Foo.prototype.getField = function() { return this.field; };" +
"/** @constructor */" +
"function Bar() {}" +
"/** @type {string} */ Bar.prototype.field;" +
"Bar.prototype.getField = function() { return this.field; };" +
"new Foo().getField();" +
"new Bar().getField();";
testSame(options, code);
options.inlineGetters = true;
test(options, code,
"function Foo() {}" +
"Foo.prototype.field;" +
"Foo.prototype.getField = function() { return this.field; };" +
"function Bar() {}" +
"Bar.prototype.field;" +
"Bar.prototype.getField = function() { return this.field; };" +
"new Foo().field;" +
"new Bar().field;");
options.checkTypes = true;
options.ambiguateProperties = true;
// Propagating the wrong type information may cause ambiguate properties
// to generate bad code.
testSame(options, code);
}
public void testInlineVariables() {
CompilerOptions options = createCompilerOptions();
String code = "function foo() {} var x = 3; foo(x);";
testSame(options, code);
options.inlineVariables = true;
test(options, code, "(function foo() {})(3);");
options.propertyRenaming = PropertyRenamingPolicy.HEURISTIC;
test(options, code, DefaultPassConfig.CANNOT_USE_PROTOTYPE_AND_VAR);
}
public void testInlineConstants() {
CompilerOptions options = createCompilerOptions();
String code = "function foo() {} var x = 3; foo(x); var YYY = 4; foo(YYY);";
testSame(options, code);
options.inlineConstantVars = true;
test(options, code, "function foo() {} var x = 3; foo(x); foo(4);");
}
public void testMinimizeExits() {
CompilerOptions options = createCompilerOptions();
String code =
"function f() {" +
" if (window.foo) return; window.h(); " +
"}";
testSame(options, code);
options.foldConstants = true;
test(
options, code,
"function f() {" +
" window.foo || window.h(); " +
"}");
}
public void testFoldConstants() {
CompilerOptions options = createCompilerOptions();
String code = "if (true) { window.foo(); }";
testSame(options, code);
options.foldConstants = true;
test(options, code, "window.foo();");
}
public void testRemoveUnreachableCode() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { return; f(); }";
testSame(options, code);
options.removeDeadCode = true;
test(options, code, "function f() {}");
}
public void testRemoveUnusedPrototypeProperties1() {
CompilerOptions options = createCompilerOptions();
String code = "function Foo() {} " +
"Foo.prototype.bar = function() { return new Foo(); };";
testSame(options, code);
options.removeUnusedPrototypeProperties = true;
test(options, code, "function Foo() {}");
}
public void testRemoveUnusedPrototypeProperties2() {
CompilerOptions options = createCompilerOptions();
String code = "function Foo() {} " +
"Foo.prototype.bar = function() { return new Foo(); };" +
"function f(x) { x.bar(); }";
testSame(options, code);
options.removeUnusedPrototypeProperties = true;
testSame(options, code);
options.removeUnusedVars = true;
test(options, code, "");
}
public void testSmartNamePass() {
CompilerOptions options = createCompilerOptions();
String code = "function Foo() { this.bar(); } " +
"Foo.prototype.bar = function() { return Foo(); };";
testSame(options, code);
options.smartNameRemoval = true;
test(options, code, "");
}
public void testDeadAssignmentsElimination() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { var x = 3; 4; x = 5; return x; } f(); ";
testSame(options, code);
options.deadAssignmentElimination = true;
testSame(options, code);
options.removeUnusedVars = true;
test(options, code, "function f() { var x = 3; 4; x = 5; return x; } f();");
}
public void testInlineFunctions() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { return 3; } f(); ";
testSame(options, code);
options.inlineFunctions = true;
test(options, code, "3;");
}
public void testRemoveUnusedVars1() {
CompilerOptions options = createCompilerOptions();
String code = "function f(x) {} f();";
testSame(options, code);
options.removeUnusedVars = true;
test(options, code, "function f() {} f();");
}
public void testRemoveUnusedVars2() {
CompilerOptions options = createCompilerOptions();
String code = "(function f(x) {})();var g = function() {}; g();";
testSame(options, code);
options.removeUnusedVars = true;
test(options, code, "(function() {})();var g = function() {}; g();");
options.anonymousFunctionNaming = AnonymousFunctionNamingPolicy.UNMAPPED;
test(options, code, "(function f() {})();var g = function $g$() {}; g();");
}
public void testCrossModuleCodeMotion() {
CompilerOptions options = createCompilerOptions();
String[] code = new String[] {
"var x = 1;",
"x;",
};
testSame(options, code);
options.crossModuleCodeMotion = true;
test(options, code, new String[] {
"",
"var x = 1; x;",
});
}
public void testCrossModuleMethodMotion() {
CompilerOptions options = createCompilerOptions();
String[] code = new String[] {
"var Foo = function() {}; Foo.prototype.bar = function() {};" +
"var x = new Foo();",
"x.bar();",
};
testSame(options, code);
options.crossModuleMethodMotion = true;
test(options, code, new String[] {
CrossModuleMethodMotion.STUB_DECLARATIONS +
"var Foo = function() {};" +
"Foo.prototype.bar=JSCompiler_stubMethod(0); var x=new Foo;",
"Foo.prototype.bar=JSCompiler_unstubMethod(0,function(){}); x.bar()",
});
}
public void testFlowSensitiveInlineVariables1() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { var x = 3; x = 5; return x; }";
testSame(options, code);
options.flowSensitiveInlineVariables = true;
test(options, code, "function f() { var x = 3; return 5; }");
String unusedVar = "function f() { var x; x = 5; return x; } f()";
test(options, unusedVar, "function f() { var x; return 5; } f()");
options.removeUnusedVars = true;
test(options, unusedVar, "function f() { return 5; } f()");
}
public void testFlowSensitiveInlineVariables2() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.SIMPLE_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
test(options,
"function f () {\n" +
" var ab = 0;\n" +
" ab += '-';\n" +
" alert(ab);\n" +
"}",
"function f () {\n" +
" alert('0-');\n" +
"}");
}
public void testCollapseAnonymousFunctions() {
CompilerOptions options = createCompilerOptions();
String code = "var f = function() {};";
testSame(options, code);
options.collapseAnonymousFunctions = true;
test(options, code, "function f() {}");
}
public void testMoveFunctionDeclarations() {
CompilerOptions options = createCompilerOptions();
String code = "var x = f(); function f() { return 3; }";
testSame(options, code);
options.moveFunctionDeclarations = true;
test(options, code, "function f() { return 3; } var x = f();");
}
public void testNameAnonymousFunctions() {
CompilerOptions options = createCompilerOptions();
String code = "var f = function() {};";
testSame(options, code);
options.anonymousFunctionNaming = AnonymousFunctionNamingPolicy.MAPPED;
test(options, code, "var f = function $() {}");
assertNotNull(lastCompiler.getResult().namedAnonFunctionMap);
options.anonymousFunctionNaming = AnonymousFunctionNamingPolicy.UNMAPPED;
test(options, code, "var f = function $f$() {}");
assertNull(lastCompiler.getResult().namedAnonFunctionMap);
}
public void testExtractPrototypeMemberDeclarations() {
CompilerOptions options = createCompilerOptions();
String code = "var f = function() {};";
String expected = "var a; var b = function() {}; a = b.prototype;";
for (int i = 0; i < 10; i++) {
code += "f.prototype.a = " + i + ";";
expected += "a.a = " + i + ";";
}
testSame(options, code);
options.extractPrototypeMemberDeclarations = true;
options.variableRenaming = VariableRenamingPolicy.ALL;
test(options, code, expected);
options.propertyRenaming = PropertyRenamingPolicy.HEURISTIC;
options.variableRenaming = VariableRenamingPolicy.OFF;
testSame(options, code);
}
public void testDevirtualizationAndExtractPrototypeMemberDeclarations() {
CompilerOptions options = createCompilerOptions();
options.devirtualizePrototypeMethods = true;
options.collapseAnonymousFunctions = true;
options.extractPrototypeMemberDeclarations = true;
options.variableRenaming = VariableRenamingPolicy.ALL;
String code = "var f = function() {};";
String expected = "var a; function b() {} a = b.prototype;";
for (int i = 0; i < 10; i++) {
code += "f.prototype.argz = function() {arguments};";
code += "f.prototype.devir" + i + " = function() {};";
char letter = (char) ('d' + i);
expected += "a.argz = function() {arguments};";
expected += "function " + letter + "(c){}";
}
code += "var F = new f(); F.argz();";
expected += "var n = new b(); n.argz();";
for (int i = 0; i < 10; i++) {
code += "F.devir" + i + "();";
char letter = (char) ('d' + i);
expected += letter + "(n);";
}
test(options, code, expected);
}
public void testCoalesceVariableNames() {
CompilerOptions options = createCompilerOptions();
String code = "function f() {var x = 3; var y = x; var z = y; return z;}";
testSame(options, code);
options.coalesceVariableNames = true;
test(options, code,
"function f() {var x = 3; x = x; x = x; return x;}");
}
public void testPropertyRenaming() {
CompilerOptions options = createCompilerOptions();
options.propertyAffinity = true;
String code =
"function f() { return this.foo + this['bar'] + this.Baz; }" +
"f.prototype.bar = 3; f.prototype.Baz = 3;";
String heuristic =
"function f() { return this.foo + this['bar'] + this.a; }" +
"f.prototype.bar = 3; f.prototype.a = 3;";
String aggHeuristic =
"function f() { return this.foo + this['b'] + this.a; } " +
"f.prototype.b = 3; f.prototype.a = 3;";
String all =
"function f() { return this.b + this['bar'] + this.a; }" +
"f.prototype.c = 3; f.prototype.a = 3;";
testSame(options, code);
options.propertyRenaming = PropertyRenamingPolicy.HEURISTIC;
test(options, code, heuristic);
options.propertyRenaming = PropertyRenamingPolicy.AGGRESSIVE_HEURISTIC;
test(options, code, aggHeuristic);
options.propertyRenaming = PropertyRenamingPolicy.ALL_UNQUOTED;
test(options, code, all);
}
public void testConvertToDottedProperties() {
CompilerOptions options = createCompilerOptions();
String code =
"function f() { return this['bar']; } f.prototype.bar = 3;";
String expected =
"function f() { return this.bar; } f.prototype.a = 3;";
testSame(options, code);
options.convertToDottedProperties = true;
options.propertyRenaming = PropertyRenamingPolicy.ALL_UNQUOTED;
test(options, code, expected);
}
public void testRewriteFunctionExpressions() {
CompilerOptions options = createCompilerOptions();
String code = "var a = function() {};";
String expected = "function JSCompiler_emptyFn(){return function(){}} " +
"var a = JSCompiler_emptyFn();";
for (int i = 0; i < 10; i++) {
code += "a = function() {};";
expected += "a = JSCompiler_emptyFn();";
}
testSame(options, code);
options.rewriteFunctionExpressions = true;
test(options, code, expected);
}
public void testAliasAllStrings() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { return 'a'; }";
String expected = "var $$S_a = 'a'; function f() { return $$S_a; }";
testSame(options, code);
options.aliasAllStrings = true;
test(options, code, expected);
}
public void testAliasExterns() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { return window + window + window + window; }";
String expected = "var GLOBAL_window = window;" +
"function f() { return GLOBAL_window + GLOBAL_window + " +
" GLOBAL_window + GLOBAL_window; }";
testSame(options, code);
options.aliasExternals = true;
test(options, code, expected);
}
public void testAliasKeywords() {
CompilerOptions options = createCompilerOptions();
String code =
"function f() { return true + true + true + true + true + true; }";
String expected = "var JSCompiler_alias_TRUE = true;" +
"function f() { return JSCompiler_alias_TRUE + " +
" JSCompiler_alias_TRUE + JSCompiler_alias_TRUE + " +
" JSCompiler_alias_TRUE + JSCompiler_alias_TRUE + " +
" JSCompiler_alias_TRUE; }";
testSame(options, code);
options.aliasKeywords = true;
test(options, code, expected);
}
public void testRenameVars1() {
CompilerOptions options = createCompilerOptions();
String code =
"var abc = 3; function f() { var xyz = 5; return abc + xyz; }";
String local = "var abc = 3; function f() { var a = 5; return abc + a; }";
String all = "var a = 3; function c() { var b = 5; return a + b; }";
testSame(options, code);
options.variableRenaming = VariableRenamingPolicy.LOCAL;
test(options, code, local);
options.variableRenaming = VariableRenamingPolicy.ALL;
test(options, code, all);
options.reserveRawExports = true;
}
public void testRenameVars2() {
CompilerOptions options = createCompilerOptions();
options.variableRenaming = VariableRenamingPolicy.ALL;
String code = "var abc = 3; function f() { window['a'] = 5; }";
String noexport = "var a = 3; function b() { window['a'] = 5; }";
String export = "var b = 3; function c() { window['a'] = 5; }";
options.reserveRawExports = false;
test(options, code, noexport);
options.reserveRawExports = true;
test(options, code, export);
}
public void testShadowVaribles() {
CompilerOptions options = createCompilerOptions();
options.variableRenaming = VariableRenamingPolicy.LOCAL;
options.shadowVariables = true;
String code = "var f = function(x) { return function(y) {}}";
String expected = "var f = function(a) { return function(a) {}}";
test(options, code, expected);
}
public void testRenameLabels() {
CompilerOptions options = createCompilerOptions();
String code = "longLabel: while (true) { break longLabel; }";
String expected = "a: while (true) { break a; }";
testSame(options, code);
options.labelRenaming = true;
test(options, code, expected);
}
public void testBadBreakStatementInIdeMode() {
// Ensure that type-checking doesn't crash, even if the CFG is malformed.
// This can happen in IDE mode.
CompilerOptions options = createCompilerOptions();
options.ideMode = true;
options.checkTypes = true;
test(options,
"function f() { try { } catch(e) { break; } }",
RhinoErrorReporter.PARSE_ERROR);
}
public void testIssue63SourceMap() {
CompilerOptions options = createCompilerOptions();
String code = "var a;";
options.skipAllPasses = true;
options.sourceMapOutputPath = "./src.map";
Compiler compiler = compile(options, code);
compiler.toSource();
}
public void testRegExp1() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = "/(a)/.test(\"a\");";
testSame(options, code);
options.computeFunctionSideEffects = true;
String expected = "";
test(options, code, expected);
}
public void testRegExp2() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = "/(a)/.test(\"a\");var a = RegExp.$1";
testSame(options, code);
options.computeFunctionSideEffects = true;
test(options, code, CheckRegExp.REGEXP_REFERENCE);
options.setWarningLevel(DiagnosticGroups.CHECK_REGEXP, CheckLevel.OFF);
testSame(options, code);
}
public void testFoldLocals1() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
// An external object, whose constructor has no side-effects,
// and whose method "go" only modifies the object.
String code = "new Widget().go();";
testSame(options, code);
options.computeFunctionSideEffects = true;
test(options, code, "");
}
public void testFoldLocals2() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
options.checkTypes = true;
// An external function that returns a local object that the
// method "go" that only modifies the object.
String code = "widgetToken().go();";
testSame(options, code);
options.computeFunctionSideEffects = true;
test(options, code, "widgetToken()");
}
public void testFoldLocals3() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
// A function "f" who returns a known local object, and a method that
// modifies only modifies that.
String definition = "function f(){return new Widget()}";
String call = "f().go();";
String code = definition + call;
testSame(options, code);
options.computeFunctionSideEffects = true;
// BROKEN
//test(options, code, definition);
testSame(options, code);
}
public void testFoldLocals4() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = "/** @constructor */\n"
+ "function InternalWidget(){this.x = 1;}"
+ "InternalWidget.prototype.internalGo = function (){this.x = 2};"
+ "new InternalWidget().internalGo();";
testSame(options, code);
options.computeFunctionSideEffects = true;
String optimized = ""
+ "function InternalWidget(){this.x = 1;}"
+ "InternalWidget.prototype.internalGo = function (){this.x = 2};";
test(options, code, optimized);
}
public void testFoldLocals5() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = ""
+ "function fn(){var a={};a.x={};return a}"
+ "fn().x.y = 1;";
// "fn" returns a unescaped local object, we should be able to fold it,
// but we don't currently.
String result = ""
+ "function fn(){var a={x:{}};return a}"
+ "fn().x.y = 1;";
test(options, code, result);
options.computeFunctionSideEffects = true;
test(options, code, result);
}
public void testFoldLocals6() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = ""
+ "function fn(){return {}}"
+ "fn().x.y = 1;";
testSame(options, code);
options.computeFunctionSideEffects = true;
testSame(options, code);
}
public void testFoldLocals7() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = ""
+ "function InternalWidget(){return [];}"
+ "Array.prototype.internalGo = function (){this.x = 2};"
+ "InternalWidget().internalGo();";
testSame(options, code);
options.computeFunctionSideEffects = true;
String optimized = ""
+ "function InternalWidget(){return [];}"
+ "Array.prototype.internalGo = function (){this.x = 2};";
test(options, code, optimized);
}
public void testVarDeclarationsIntoFor() {
CompilerOptions options = createCompilerOptions();
options.collapseVariableDeclarations = false;
String code = "var a = 1; for (var b = 2; ;) {}";
testSame(options, code);
options.collapseVariableDeclarations = false;
test(options, code, "for (var a = 1, b = 2; ;) {}");
}
public void testExploitAssigns() {
CompilerOptions options = createCompilerOptions();
options.collapseVariableDeclarations = false;
String code = "a = 1; b = a; c = b";
testSame(options, code);
options.collapseVariableDeclarations = true;
test(options, code, "c=b=a=1");
}
public void testRecoverOnBadExterns() throws Exception {
// This test is for a bug in a very narrow set of circumstances:
// 1) externs validation has to be off.
// 2) aliasExternals has to be on.
// 3) The user has to reference a "normal" variable in externs.
// This case is handled at checking time by injecting a
// synthetic extern variable, and adding a "@suppress {duplicate}" to
// the normal code at compile time. But optimizations may remove that
// annotation, so we need to make sure that the variable declarations
// are de-duped before that happens.
CompilerOptions options = createCompilerOptions();
options.aliasExternals = true;
externs = new JSSourceFile[] {
JSSourceFile.fromCode("externs", "extern.foo")
};
test(options,
"var extern; " +
"function f() { return extern + extern + extern + extern; }",
"var extern; " +
"function f() { return extern + extern + extern + extern; }",
VarCheck.UNDEFINED_EXTERN_VAR_ERROR);
}
public void testDuplicateVariablesInExterns() {
CompilerOptions options = createCompilerOptions();
options.checkSymbols = true;
externs = new JSSourceFile[] {
JSSourceFile.fromCode("externs",
"var externs = {}; /** @suppress {duplicate} */ var externs = {};")
};
testSame(options, "");
}
public void testLanguageMode() {
CompilerOptions options = createCompilerOptions();
options.setLanguageIn(LanguageMode.ECMASCRIPT3);
String code = "var a = {get f(){}}";
Compiler compiler = compile(options, code);
checkUnexpectedErrorsOrWarnings(compiler, 1);
assertEquals(
"JSC_PARSE_ERROR. Parse error. " +
"getters are not supported in Internet Explorer " +
"at i0 line 1 : 0",
compiler.getErrors()[0].toString());
options.setLanguageIn(LanguageMode.ECMASCRIPT5);
testSame(options, code);
options.setLanguageIn(LanguageMode.ECMASCRIPT5_STRICT);
testSame(options, code);
}
public void testLanguageMode2() {
CompilerOptions options = createCompilerOptions();
options.setLanguageIn(LanguageMode.ECMASCRIPT3);
options.setWarningLevel(DiagnosticGroups.ES5_STRICT, CheckLevel.OFF);
String code = "var a = 2; delete a;";
testSame(options, code);
options.setLanguageIn(LanguageMode.ECMASCRIPT5);
testSame(options, code);
options.setLanguageIn(LanguageMode.ECMASCRIPT5_STRICT);
test(options,
code,
code,
StrictModeCheck.DELETE_VARIABLE);
}
public void testIssue598() {
CompilerOptions options = createCompilerOptions();
options.setLanguageIn(LanguageMode.ECMASCRIPT5_STRICT);
WarningLevel.VERBOSE.setOptionsForWarningLevel(options);
options.setLanguageIn(LanguageMode.ECMASCRIPT5);
String code =
"'use strict';\n" +
"function App() {}\n" +
"App.prototype = {\n" +
" get appData() { return this.appData_; },\n" +
" set appData(data) { this.appData_ = data; }\n" +
"};";
Compiler compiler = compile(options, code);
testSame(options, code);
}
public void testCoaleseVariables() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = false;
options.coalesceVariableNames = true;
String code =
"function f(a) {" +
" if (a) {" +
" return a;" +
" } else {" +
" var b = a;" +
" return b;" +
" }" +
" return a;" +
"}";
String expected =
"function f(a) {" +
" if (a) {" +
" return a;" +
" } else {" +
" a = a;" +
" return a;" +
" }" +
" return a;" +
"}";
test(options, code, expected);
options.foldConstants = true;
options.coalesceVariableNames = false;
code =
"function f(a) {" +
" if (a) {" +
" return a;" +
" } else {" +
" var b = a;" +
" return b;" +
" }" +
" return a;" +
"}";
expected =
"function f(a) {" +
" if (!a) {" +
" var b = a;" +
" return b;" +
" }" +
" return a;" +
"}";
test(options, code, expected);
options.foldConstants = true;
options.coalesceVariableNames = true;
expected =
"function f(a) {" +
" return a;" +
"}";
test(options, code, expected);
}
public void testLateStatementFusion() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
test(options,
"while(a){a();if(b){b();b()}}",
"for(;a;)a(),b&&(b(),b())");
}
public void testLateConstantReordering() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
test(options,
"if (x < 1 || x > 1 || 1 < x || 1 > x) { alert(x) }",
" (1 > x || 1 < x || 1 < x || 1 > x) && alert(x) ");
}
public void testsyntheticBlockOnDeadAssignments() {
CompilerOptions options = createCompilerOptions();
options.deadAssignmentElimination = true;
options.removeUnusedVars = true;
options.syntheticBlockStartMarker = "START";
options.syntheticBlockEndMarker = "END";
test(options, "var x; x = 1; START(); x = 1;END();x()",
"var x; x = 1;{START();{x = 1}END()}x()");
}
public void testBug4152835() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
options.syntheticBlockStartMarker = "START";
options.syntheticBlockEndMarker = "END";
test(options, "START();END()", "{START();{}END()}");
}
public void testBug5786871() {
CompilerOptions options = createCompilerOptions();
options.ideMode = true;
test(options, "function () {}", RhinoErrorReporter.PARSE_ERROR);
}
public void testIssue378() {
CompilerOptions options = createCompilerOptions();
options.inlineVariables = true;
options.flowSensitiveInlineVariables = true;
testSame(options, "function f(c) {var f = c; arguments[0] = this;" +
" f.apply(this, arguments); return this;}");
}
public void testIssue550() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.SIMPLE_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
options.foldConstants = true;
options.inlineVariables = true;
options.flowSensitiveInlineVariables = true;
test(options,
"function f(h) {\n" +
" var a = h;\n" +
" a = a + 'x';\n" +
" a = a + 'y';\n" +
" return a;\n" +
"}",
"function f(a) {return a + 'xy'}");
}
public void testIssue284() {
CompilerOptions options = createCompilerOptions();
options.smartNameRemoval = true;
test(options,
"var goog = {};" +
"goog.inherits = function(x, y) {};" +
"var ns = {};" +
"/** @constructor */" +
"ns.PageSelectionModel = function() {};" +
"/** @constructor */" +
"ns.PageSelectionModel.FooEvent = function() {};" +
"/** @constructor */" +
"ns.PageSelectionModel.SelectEvent = function() {};" +
"goog.inherits(ns.PageSelectionModel.ChangeEvent," +
" ns.PageSelectionModel.FooEvent);",
"");
}
public void testCodingConvention() {
Compiler compiler = new Compiler();
compiler.initOptions(new CompilerOptions());
assertEquals(
compiler.getCodingConvention().getClass().toString(),
ClosureCodingConvention.class.toString());
}
public void testJQueryStringSplitLoops() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
test(options,
"var x=['1','2','3','4','5','6','7']",
"var x='1,2,3,4,5,6,7'.split(',')");
options = createCompilerOptions();
options.foldConstants = true;
options.computeFunctionSideEffects = false;
options.removeUnusedVars = true;
// If we do splits too early, it would add a sideeffect to x.
test(options,
"var x=['1','2','3','4','5','6','7']",
"");
}
public void testAlwaysRunSafetyCheck() {
CompilerOptions options = createCompilerOptions();
options.checkSymbols = false;
options.customPasses = ArrayListMultimap.create();
options.customPasses.put(
CustomPassExecutionTime.BEFORE_OPTIMIZATIONS,
new CompilerPass() {
@Override public void process(Node externs, Node root) {
Node var = root.getLastChild().getFirstChild();
assertEquals(Token.VAR, var.getType());
var.detachFromParent();
}
});
try {
test(options,
"var x = 3; function f() { return x + z; }",
"function f() { return x + z; }");
fail("Expected runtime exception");
} catch (RuntimeException e) {
assertTrue(e.getMessage().indexOf("Unexpected variable x") != -1);
}
}
public void testSuppressEs5StrictWarning() {
CompilerOptions options = createCompilerOptions();
options.setWarningLevel(DiagnosticGroups.ES5_STRICT, CheckLevel.WARNING);
testSame(options,
"/** @suppress{es5Strict} */\n" +
"function f() { var arguments; }");
}
public void testCheckProvidesWarning() {
CompilerOptions options = createCompilerOptions();
options.setWarningLevel(DiagnosticGroups.CHECK_PROVIDES, CheckLevel.WARNING);
options.setCheckProvides(CheckLevel.WARNING);
test(options,
"/** @constructor */\n" +
"function f() { var arguments; }",
DiagnosticType.warning("JSC_MISSING_PROVIDE", "missing goog.provide(''{0}'')"));
}
public void testSuppressCheckProvidesWarning() {
CompilerOptions options = createCompilerOptions();
options.setWarningLevel(DiagnosticGroups.CHECK_PROVIDES, CheckLevel.WARNING);
options.setCheckProvides(CheckLevel.WARNING);
testSame(options,
"/** @constructor\n" +
" * @suppress{checkProvides} */\n" +
"function f() { var arguments; }");
}
public void testRenamePrefixNamespace() {
String code =
"var x = {}; x.FOO = 5; x.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.collapseProperties = true;
options.renamePrefixNamespace = "_";
test(options, code, "_.x$FOO = 5; _.x$bar = 3;");
}
public void testRenamePrefixNamespaceActivatesMoveFunctionDeclarations() {
CompilerOptions options = createCompilerOptions();
String code = "var x = f; function f() { return 3; }";
testSame(options, code);
assertFalse(options.moveFunctionDeclarations);
options.renamePrefixNamespace = "_";
test(options, code, "_.f = function() { return 3; }; _.x = _.f;");
}
public void testBrokenNameSpace() {
CompilerOptions options = createCompilerOptions();
String code = "var goog; goog.provide('i.am.on.a.Horse');" +
"i.am.on.a.Horse = function() {};" +
"i.am.on.a.Horse.prototype.x = function() {};" +
"i.am.on.a.Boat.prototype.y = function() {}";
options.closurePass = true;
options.collapseProperties = true;
options.smartNameRemoval = true;
test(options, code, "");
}
public void testNamelessParameter() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.ADVANCED_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
String code =
"var impl_0;" +
"$load($init());" +
"function $load(){" +
" window['f'] = impl_0;" +
"}" +
"function $init() {" +
" impl_0 = {};" +
"}";
String result =
"window.f = {};";
test(options, code, result);
}
public void testHiddenSideEffect() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.ADVANCED_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
options.setAliasExternals(true);
String code =
"window.offsetWidth;";
String result =
"window.offsetWidth;";
test(options, code, result);
}
public void testNegativeZero() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.ADVANCED_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
test(options,
"function bar(x) { return x; }\n" +
"function foo(x) { print(x / bar(0));\n" +
" print(x / bar(-0)); }\n" +
"foo(3);",
"print(3/0);print(3/-0);");
}
private void testSame(CompilerOptions options, String original) {
testSame(options, new String[] { original });
}
private void testSame(CompilerOptions options, String[] original) {
test(options, original, original);
}
/**
* Asserts that when compiling with the given compiler options,
* {@code original} is transformed into {@code compiled}.
*/
private void test(CompilerOptions options,
String original, String compiled) {
test(options, new String[] { original }, new String[] { compiled });
}
/**
* Asserts that when compiling with the given compiler options,
* {@code original} is transformed into {@code compiled}.
*/
private void test(CompilerOptions options,
String[] original, String[] compiled) {
Compiler compiler = compile(options, original);
assertEquals("Expected no warnings or errors\n" +
"Errors: \n" + Joiner.on("\n").join(compiler.getErrors()) +
"Warnings: \n" + Joiner.on("\n").join(compiler.getWarnings()),
0, compiler.getErrors().length + compiler.getWarnings().length);
Node root = compiler.getRoot().getLastChild();
Node expectedRoot = parse(compiled, options);
String explanation = expectedRoot.checkTreeEquals(root);
assertNull("\nExpected: " + compiler.toSource(expectedRoot) +
"\nResult: " + compiler.toSource(root) +
"\n" + explanation, explanation);
}
/**
* Asserts that when compiling with the given compiler options,
* there is an error or warning.
*/
private void test(CompilerOptions options,
String original, DiagnosticType warning) {
test(options, new String[] { original }, warning);
}
private void test(CompilerOptions options,
String original, String compiled, DiagnosticType warning) {
test(options, new String[] { original }, new String[] { compiled },
warning);
}
private void test(CompilerOptions options,
String[] original, DiagnosticType warning) {
test(options, original, null, warning);
}
/**
* Asserts that when compiling with the given compiler options,
* there is an error or warning.
*/
private void test(CompilerOptions options,
String[] original, String[] compiled, DiagnosticType warning) {
Compiler compiler = compile(options, original);
checkUnexpectedErrorsOrWarnings(compiler, 1);
assertEquals("Expected exactly one warning or error",
1, compiler.getErrors().length + compiler.getWarnings().length);
if (compiler.getErrors().length > 0) {
assertEquals(warning, compiler.getErrors()[0].getType());
} else {
assertEquals(warning, compiler.getWarnings()[0].getType());
}
if (compiled != null) {
Node root = compiler.getRoot().getLastChild();
Node expectedRoot = parse(compiled, options);
String explanation = expectedRoot.checkTreeEquals(root);
assertNull("\nExpected: " + compiler.toSource(expectedRoot) +
"\nResult: " + compiler.toSource(root) +
"\n" + explanation, explanation);
}
}
private void checkUnexpectedErrorsOrWarnings(
Compiler compiler, int expected) {
int actual = compiler.getErrors().length + compiler.getWarnings().length;
if (actual != expected) {
String msg = "";
for (JSError err : compiler.getErrors()) {
msg += "Error:" + err.toString() + "\n";
}
for (JSError err : compiler.getWarnings()) {
msg += "Warning:" + err.toString() + "\n";
}
assertEquals("Unexpected warnings or errors.\n "+ msg,
expected, actual);
}
}
private Compiler compile(CompilerOptions options, String original) {
return compile(options, new String[] { original });
}
private Compiler compile(CompilerOptions options, String[] original) {
Compiler compiler = lastCompiler = new Compiler();
JSSourceFile[] inputs = new JSSourceFile[original.length];
for (int i = 0; i < original.length; i++) {
inputs[i] = JSSourceFile.fromCode("input" + i, original[i]);
}
compiler.compile(
externs, CompilerTestCase.createModuleChain(original), options);
return compiler;
}
private Node parse(String[] original, CompilerOptions options) {
Compiler compiler = new Compiler();
JSSourceFile[] inputs = new JSSourceFile[original.length];
for (int i = 0; i < inputs.length; i++) {
inputs[i] = JSSourceFile.fromCode("input" + i, original[i]);
}
compiler.init(externs, inputs, options);
checkUnexpectedErrorsOrWarnings(compiler, 0);
Node all = compiler.parseInputs();
checkUnexpectedErrorsOrWarnings(compiler, 0);
Node n = all.getLastChild();
Node externs = all.getFirstChild();
(new CreateSyntheticBlocks(
compiler, "synStart", "synEnd")).process(externs, n);
(new Normalize(compiler, false)).process(externs, n);
(MakeDeclaredNamesUnique.getContextualRenameInverter(compiler)).process(
externs, n);
(new Denormalize(compiler)).process(externs, n);
return n;
}
/** Creates a CompilerOptions object with google coding conventions. */
private CompilerOptions createCompilerOptions() {
CompilerOptions options = new CompilerOptions();
options.setCodingConvention(new GoogleCodingConvention());
return options;
}
} |
||
public void testMinpackJennrichSampson() {
minpackTest(new JennrichSampsonFunction(10, new double[] { 0.3, 0.4 },
64.5856498144943, 11.1517793413499,
new double[] {
// 0.2578330049, 0.257829976764542
0.2578199266368004, 0.25782997676455244
}), false);
} | org.apache.commons.math.optimization.general.MinpackTest::testMinpackJennrichSampson | src/test/java/org/apache/commons/math/optimization/general/MinpackTest.java | 330 | src/test/java/org/apache/commons/math/optimization/general/MinpackTest.java | testMinpackJennrichSampson | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.math.optimization.general;
import java.io.Serializable;
import java.util.Arrays;
import junit.framework.TestCase;
import org.apache.commons.math.FunctionEvaluationException;
import org.apache.commons.math.analysis.DifferentiableMultivariateVectorialFunction;
import org.apache.commons.math.analysis.MultivariateMatrixFunction;
import org.apache.commons.math.optimization.OptimizationException;
import org.apache.commons.math.optimization.VectorialPointValuePair;
/**
* <p>Some of the unit tests are re-implementations of the MINPACK <a
* href="http://www.netlib.org/minpack/ex/file17">file17</a> and <a
* href="http://www.netlib.org/minpack/ex/file22">file22</a> test files.
* The redistribution policy for MINPACK is available <a
* href="http://www.netlib.org/minpack/disclaimer">here</a>, for
* convenience, it is reproduced below.</p>
* <table border="0" width="80%" cellpadding="10" align="center" bgcolor="#E0E0E0">
* <tr><td>
* Minpack Copyright Notice (1999) University of Chicago.
* All rights reserved
* </td></tr>
* <tr><td>
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
* <ol>
* <li>Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.</li>
* <li>Redistributions in binary form must reproduce the above
* copyright notice, this list of conditions and the following
* disclaimer in the documentation and/or other materials provided
* with the distribution.</li>
* <li>The end-user documentation included with the redistribution, if any,
* must include the following acknowledgment:
* <code>This product includes software developed by the University of
* Chicago, as Operator of Argonne National Laboratory.</code>
* Alternately, this acknowledgment may appear in the software itself,
* if and wherever such third-party acknowledgments normally appear.</li>
* <li><strong>WARRANTY DISCLAIMER. THE SOFTWARE IS SUPPLIED "AS IS"
* WITHOUT WARRANTY OF ANY KIND. THE COPYRIGHT HOLDER, THE
* UNITED STATES, THE UNITED STATES DEPARTMENT OF ENERGY, AND
* THEIR EMPLOYEES: (1) DISCLAIM ANY WARRANTIES, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO ANY IMPLIED WARRANTIES
* OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE, TITLE
* OR NON-INFRINGEMENT, (2) DO NOT ASSUME ANY LEGAL LIABILITY
* OR RESPONSIBILITY FOR THE ACCURACY, COMPLETENESS, OR
* USEFULNESS OF THE SOFTWARE, (3) DO NOT REPRESENT THAT USE OF
* THE SOFTWARE WOULD NOT INFRINGE PRIVATELY OWNED RIGHTS, (4)
* DO NOT WARRANT THAT THE SOFTWARE WILL FUNCTION
* UNINTERRUPTED, THAT IT IS ERROR-FREE OR THAT ANY ERRORS WILL
* BE CORRECTED.</strong></li>
* <li><strong>LIMITATION OF LIABILITY. IN NO EVENT WILL THE COPYRIGHT
* HOLDER, THE UNITED STATES, THE UNITED STATES DEPARTMENT OF
* ENERGY, OR THEIR EMPLOYEES: BE LIABLE FOR ANY INDIRECT,
* INCIDENTAL, CONSEQUENTIAL, SPECIAL OR PUNITIVE DAMAGES OF
* ANY KIND OR NATURE, INCLUDING BUT NOT LIMITED TO LOSS OF
* PROFITS OR LOSS OF DATA, FOR ANY REASON WHATSOEVER, WHETHER
* SUCH LIABILITY IS ASSERTED ON THE BASIS OF CONTRACT, TORT
* (INCLUDING NEGLIGENCE OR STRICT LIABILITY), OR OTHERWISE,
* EVEN IF ANY OF SAID PARTIES HAS BEEN WARNED OF THE
* POSSIBILITY OF SUCH LOSS OR DAMAGES.</strong></li>
* <ol></td></tr>
* </table>
* @author Argonne National Laboratory. MINPACK project. March 1980 (original fortran minpack tests)
* @author Burton S. Garbow (original fortran minpack tests)
* @author Kenneth E. Hillstrom (original fortran minpack tests)
* @author Jorge J. More (original fortran minpack tests)
* @author Luc Maisonobe (non-minpack tests and minpack tests Java translation)
*/
public class MinpackTest extends TestCase {
public MinpackTest(String name) {
super(name);
}
public void testMinpackLinearFullRank() {
minpackTest(new LinearFullRankFunction(10, 5, 1.0,
5.0, 2.23606797749979), false);
minpackTest(new LinearFullRankFunction(50, 5, 1.0,
8.06225774829855, 6.70820393249937), false);
}
public void testMinpackLinearRank1() {
minpackTest(new LinearRank1Function(10, 5, 1.0,
291.521868819476, 1.4638501094228), false);
minpackTest(new LinearRank1Function(50, 5, 1.0,
3101.60039334535, 3.48263016573496), false);
}
public void testMinpackLinearRank1ZeroColsAndRows() {
minpackTest(new LinearRank1ZeroColsAndRowsFunction(10, 5, 1.0), false);
minpackTest(new LinearRank1ZeroColsAndRowsFunction(50, 5, 1.0), false);
}
public void testMinpackRosenbrok() {
minpackTest(new RosenbrockFunction(new double[] { -1.2, 1.0 },
Math.sqrt(24.2)), false);
minpackTest(new RosenbrockFunction(new double[] { -12.0, 10.0 },
Math.sqrt(1795769.0)), false);
minpackTest(new RosenbrockFunction(new double[] { -120.0, 100.0 },
11.0 * Math.sqrt(169000121.0)), false);
}
public void testMinpackHelicalValley() {
minpackTest(new HelicalValleyFunction(new double[] { -1.0, 0.0, 0.0 },
50.0), false);
minpackTest(new HelicalValleyFunction(new double[] { -10.0, 0.0, 0.0 },
102.95630140987), false);
minpackTest(new HelicalValleyFunction(new double[] { -100.0, 0.0, 0.0},
991.261822123701), false);
}
public void testMinpackPowellSingular() {
minpackTest(new PowellSingularFunction(new double[] { 3.0, -1.0, 0.0, 1.0 },
14.6628782986152), false);
minpackTest(new PowellSingularFunction(new double[] { 30.0, -10.0, 0.0, 10.0 },
1270.9838708654), false);
minpackTest(new PowellSingularFunction(new double[] { 300.0, -100.0, 0.0, 100.0 },
126887.903284750), false);
}
public void testMinpackFreudensteinRoth() {
minpackTest(new FreudensteinRothFunction(new double[] { 0.5, -2.0 },
20.0124960961895, 6.99887517584575,
new double[] {
11.4124844654993,
-0.896827913731509
}), false);
minpackTest(new FreudensteinRothFunction(new double[] { 5.0, -20.0 },
12432.833948863, 6.9988751744895,
new double[] {
11.41300466147456,
-0.896796038685959
}), false);
minpackTest(new FreudensteinRothFunction(new double[] { 50.0, -200.0 },
11426454.595762, 6.99887517242903,
new double[] {
11.412781785788564,
-0.8968051074920405
}), false);
}
public void testMinpackBard() {
minpackTest(new BardFunction(1.0, 6.45613629515967, 0.0906359603390466,
new double[] {
0.0824105765758334,
1.1330366534715,
2.34369463894115
}), false);
minpackTest(new BardFunction(10.0, 36.1418531596785, 4.17476870138539,
new double[] {
0.840666673818329,
-158848033.259565,
-164378671.653535
}), false);
minpackTest(new BardFunction(100.0, 384.114678637399, 4.17476870135969,
new double[] {
0.840666673867645,
-158946167.205518,
-164464906.857771
}), false);
}
public void testMinpackKowalikOsborne() {
minpackTest(new KowalikOsborneFunction(new double[] { 0.25, 0.39, 0.415, 0.39 },
0.0728915102882945,
0.017535837721129,
new double[] {
0.192807810476249,
0.191262653354071,
0.123052801046931,
0.136053221150517
}), false);
minpackTest(new KowalikOsborneFunction(new double[] { 2.5, 3.9, 4.15, 3.9 },
2.97937007555202,
0.032052192917937,
new double[] {
728675.473768287,
-14.0758803129393,
-32977797.7841797,
-20571594.1977912
}), false);
minpackTest(new KowalikOsborneFunction(new double[] { 25.0, 39.0, 41.5, 39.0 },
29.9590617016037,
0.0175364017658228,
new double[] {
0.192948328597594,
0.188053165007911,
0.122430604321144,
0.134575665392506
}), false);
}
public void testMinpackMeyer() {
minpackTest(new MeyerFunction(new double[] { 0.02, 4000.0, 250.0 },
41153.4665543031, 9.37794514651874,
new double[] {
0.00560963647102661,
6181.34634628659,
345.223634624144
}), false);
minpackTest(new MeyerFunction(new double[] { 0.2, 40000.0, 2500.0 },
4168216.89130846, 792.917871779501,
new double[] {
1.42367074157994e-11,
33695.7133432541,
901.268527953801
}), true);
}
public void testMinpackWatson() {
minpackTest(new WatsonFunction(6, 0.0,
5.47722557505166, 0.0478295939097601,
new double[] {
-0.0157249615083782, 1.01243488232965,
-0.232991722387673, 1.26043101102818,
-1.51373031394421, 0.99299727291842
}), false);
minpackTest(new WatsonFunction(6, 10.0,
6433.12578950026, 0.0478295939096951,
new double[] {
-0.0157251901386677, 1.01243485860105,
-0.232991545843829, 1.26042932089163,
-1.51372776706575, 0.99299573426328
}), false);
minpackTest(new WatsonFunction(6, 100.0,
674256.040605213, 0.047829593911544,
new double[] {
-0.0157247019712586, 1.01243490925658,
-0.232991922761641, 1.26043292929555,
-1.51373320452707, 0.99299901922322
}), false);
minpackTest(new WatsonFunction(9, 0.0,
5.47722557505166, 0.00118311459212420,
new double[] {
-0.153070644166722e-4, 0.999789703934597,
0.0147639634910978, 0.146342330145992,
1.00082109454817, -2.61773112070507,
4.10440313943354, -3.14361226236241,
1.05262640378759
}), false);
minpackTest(new WatsonFunction(9, 10.0,
12088.127069307, 0.00118311459212513,
new double[] {
-0.153071334849279e-4, 0.999789703941234,
0.0147639629786217, 0.146342334818836,
1.00082107321386, -2.61773107084722,
4.10440307655564, -3.14361222178686,
1.05262639322589
}), false);
minpackTest(new WatsonFunction(9, 100.0,
1269109.29043834, 0.00118311459212384,
new double[] {
-0.153069523352176e-4, 0.999789703958371,
0.0147639625185392, 0.146342341096326,
1.00082104729164, -2.61773101573645,
4.10440301427286, -3.14361218602503,
1.05262638516774
}), false);
minpackTest(new WatsonFunction(12, 0.0,
5.47722557505166, 0.217310402535861e-4,
new double[] {
-0.660266001396382e-8, 1.00000164411833,
-0.000563932146980154, 0.347820540050756,
-0.156731500244233, 1.05281515825593,
-3.24727109519451, 7.2884347837505,
-10.271848098614, 9.07411353715783,
-4.54137541918194, 1.01201187975044
}), false);
minpackTest(new WatsonFunction(12, 10.0,
19220.7589790951, 0.217310402518509e-4,
new double[] {
-0.663710223017410e-8, 1.00000164411787,
-0.000563932208347327, 0.347820540486998,
-0.156731503955652, 1.05281517654573,
-3.2472711515214, 7.28843489430665,
-10.2718482369638, 9.07411364383733,
-4.54137546533666, 1.01201188830857
}), false);
minpackTest(new WatsonFunction(12, 100.0,
2018918.04462367, 0.217310402539845e-4,
new double[] {
-0.663806046485249e-8, 1.00000164411786,
-0.000563932210324959, 0.347820540503588,
-0.156731504091375, 1.05281517718031,
-3.24727115337025, 7.28843489775302,
-10.2718482410813, 9.07411364688464,
-4.54137546660822, 1.0120118885369
}), false);
}
public void testMinpackBox3Dimensional() {
minpackTest(new Box3DimensionalFunction(10, new double[] { 0.0, 10.0, 20.0 },
32.1115837449572), false);
}
public void testMinpackJennrichSampson() {
minpackTest(new JennrichSampsonFunction(10, new double[] { 0.3, 0.4 },
64.5856498144943, 11.1517793413499,
new double[] {
// 0.2578330049, 0.257829976764542
0.2578199266368004, 0.25782997676455244
}), false);
}
public void testMinpackBrownDennis() {
minpackTest(new BrownDennisFunction(20,
new double[] { 25.0, 5.0, -5.0, -1.0 },
2815.43839161816, 292.954288244866,
new double[] {
-11.59125141003, 13.2024883984741,
-0.403574643314272, 0.236736269844604
}), false);
minpackTest(new BrownDennisFunction(20,
new double[] { 250.0, 50.0, -50.0, -10.0 },
555073.354173069, 292.954270581415,
new double[] {
-11.5959274272203, 13.2041866926242,
-0.403417362841545, 0.236771143410386
}), false);
minpackTest(new BrownDennisFunction(20,
new double[] { 2500.0, 500.0, -500.0, -100.0 },
61211252.2338581, 292.954306151134,
new double[] {
-11.5902596937374, 13.2020628854665,
-0.403688070279258, 0.236665033746463
}), false);
}
public void testMinpackChebyquad() {
minpackTest(new ChebyquadFunction(1, 8, 1.0,
1.88623796907732, 1.88623796907732,
new double[] { 0.5 }), false);
minpackTest(new ChebyquadFunction(1, 8, 10.0,
5383344372.34005, 1.88424820499951,
new double[] { 0.9817314924684 }), false);
minpackTest(new ChebyquadFunction(1, 8, 100.0,
0.118088726698392e19, 1.88424820499347,
new double[] { 0.9817314852934 }), false);
minpackTest(new ChebyquadFunction(8, 8, 1.0,
0.196513862833975, 0.0593032355046727,
new double[] {
0.0431536648587336, 0.193091637843267,
0.266328593812698, 0.499999334628884,
0.500000665371116, 0.733671406187302,
0.806908362156733, 0.956846335141266
}), false);
minpackTest(new ChebyquadFunction(9, 9, 1.0,
0.16994993465202, 0.0,
new double[] {
0.0442053461357828, 0.199490672309881,
0.23561910847106, 0.416046907892598,
0.5, 0.583953092107402,
0.764380891528940, 0.800509327690119,
0.955794653864217
}), false);
minpackTest(new ChebyquadFunction(10, 10, 1.0,
0.183747831178711, 0.0806471004038253,
new double[] {
0.0596202671753563, 0.166708783805937,
0.239171018813509, 0.398885290346268,
0.398883667870681, 0.601116332129320,
0.60111470965373, 0.760828981186491,
0.833291216194063, 0.940379732824644
}), false);
}
public void testMinpackBrownAlmostLinear() {
minpackTest(new BrownAlmostLinearFunction(10, 0.5,
16.5302162063499, 0.0,
new double[] {
0.979430303349862, 0.979430303349862,
0.979430303349862, 0.979430303349862,
0.979430303349862, 0.979430303349862,
0.979430303349862, 0.979430303349862,
0.979430303349862, 1.20569696650138
}), false);
minpackTest(new BrownAlmostLinearFunction(10, 5.0,
9765624.00089211, 0.0,
new double[] {
0.979430303349865, 0.979430303349865,
0.979430303349865, 0.979430303349865,
0.979430303349865, 0.979430303349865,
0.979430303349865, 0.979430303349865,
0.979430303349865, 1.20569696650135
}), false);
minpackTest(new BrownAlmostLinearFunction(10, 50.0,
0.9765625e17, 0.0,
new double[] {
1.0, 1.0, 1.0, 1.0, 1.0,
1.0, 1.0, 1.0, 1.0, 1.0
}), false);
minpackTest(new BrownAlmostLinearFunction(30, 0.5,
83.476044467848, 0.0,
new double[] {
0.997754216442807, 0.997754216442807,
0.997754216442807, 0.997754216442807,
0.997754216442807, 0.997754216442807,
0.997754216442807, 0.997754216442807,
0.997754216442807, 0.997754216442807,
0.997754216442807, 0.997754216442807,
0.997754216442807, 0.997754216442807,
0.997754216442807, 0.997754216442807,
0.997754216442807, 0.997754216442807,
0.997754216442807, 0.997754216442807,
0.997754216442807, 0.997754216442807,
0.997754216442807, 0.997754216442807,
0.997754216442807, 0.997754216442807,
0.997754216442807, 0.997754216442807,
0.997754216442807, 1.06737350671578
}), false);
minpackTest(new BrownAlmostLinearFunction(40, 0.5,
128.026364472323, 0.0,
new double[] {
1.00000000000002, 1.00000000000002,
1.00000000000002, 1.00000000000002,
1.00000000000002, 1.00000000000002,
1.00000000000002, 1.00000000000002,
1.00000000000002, 1.00000000000002,
1.00000000000002, 1.00000000000002,
1.00000000000002, 1.00000000000002,
1.00000000000002, 1.00000000000002,
1.00000000000002, 1.00000000000002,
1.00000000000002, 1.00000000000002,
1.00000000000002, 1.00000000000002,
1.00000000000002, 1.00000000000002,
1.00000000000002, 1.00000000000002,
1.00000000000002, 1.00000000000002,
1.00000000000002, 1.00000000000002,
1.00000000000002, 1.00000000000002,
1.00000000000002, 1.00000000000002,
0.999999999999121
}), false);
}
public void testMinpackOsborne1() {
minpackTest(new Osborne1Function(new double[] { 0.5, 1.5, -1.0, 0.01, 0.02, },
0.937564021037838, 0.00739249260904843,
new double[] {
0.375410049244025, 1.93584654543108,
-1.46468676748716, 0.0128675339110439,
0.0221227011813076
}), false);
}
public void testMinpackOsborne2() {
minpackTest(new Osborne2Function(new double[] {
1.3, 0.65, 0.65, 0.7, 0.6,
3.0, 5.0, 7.0, 2.0, 4.5, 5.5
},
1.44686540984712, 0.20034404483314,
new double[] {
1.30997663810096, 0.43155248076,
0.633661261602859, 0.599428560991695,
0.754179768272449, 0.904300082378518,
1.36579949521007, 4.82373199748107,
2.39868475104871, 4.56887554791452,
5.67534206273052
}), false);
}
private void minpackTest(MinpackFunction function, boolean exceptionExpected) {
LevenbergMarquardtOptimizer optimizer = new LevenbergMarquardtOptimizer();
optimizer.setMaxIterations(100 * (function.getN() + 1));
optimizer.setCostRelativeTolerance(Math.sqrt(2.22044604926e-16));
optimizer.setParRelativeTolerance(Math.sqrt(2.22044604926e-16));
optimizer.setOrthoTolerance(2.22044604926e-16);
// assertTrue(function.checkTheoreticalStartCost(optimizer.getRMS()));
try {
VectorialPointValuePair optimum =
optimizer.optimize(function,
function.getTarget(), function.getWeight(),
function.getStartPoint());
assertFalse(exceptionExpected);
function.checkTheoreticalMinCost(optimizer.getRMS());
function.checkTheoreticalMinParams(optimum);
} catch (OptimizationException lsse) {
assertTrue(exceptionExpected);
} catch (FunctionEvaluationException fe) {
assertTrue(exceptionExpected);
}
}
private static abstract class MinpackFunction
implements DifferentiableMultivariateVectorialFunction, Serializable {
private static final long serialVersionUID = -6209760235478794233L;
protected int n;
protected int m;
protected double[] startParams;
protected double theoreticalMinCost;
protected double[] theoreticalMinParams;
protected double costAccuracy;
protected double paramsAccuracy;
protected MinpackFunction(int m, double[] startParams,
double theoreticalMinCost, double[] theoreticalMinParams) {
this.m = m;
this.n = startParams.length;
this.startParams = startParams.clone();
this.theoreticalMinCost = theoreticalMinCost;
this.theoreticalMinParams = theoreticalMinParams;
this.costAccuracy = 1.0e-8;
this.paramsAccuracy = 1.0e-5;
}
protected static double[] buildArray(int n, double x) {
double[] array = new double[n];
Arrays.fill(array, x);
return array;
}
public double[] getTarget() {
return buildArray(m, 0.0);
}
public double[] getWeight() {
return buildArray(m, 1.0);
}
public double[] getStartPoint() {
return startParams.clone();
}
protected void setCostAccuracy(double costAccuracy) {
this.costAccuracy = costAccuracy;
}
protected void setParamsAccuracy(double paramsAccuracy) {
this.paramsAccuracy = paramsAccuracy;
}
public int getN() {
return startParams.length;
}
public void checkTheoreticalMinCost(double rms) {
double threshold = costAccuracy * (1.0 + theoreticalMinCost);
assertEquals(theoreticalMinCost, Math.sqrt(m) * rms, threshold);
}
public void checkTheoreticalMinParams(VectorialPointValuePair optimum) {
double[] params = optimum.getPointRef();
if (theoreticalMinParams != null) {
for (int i = 0; i < theoreticalMinParams.length; ++i) {
double mi = theoreticalMinParams[i];
double vi = params[i];
assertEquals(mi, vi, paramsAccuracy * (1.0 + Math.abs(mi)));
}
}
}
public MultivariateMatrixFunction jacobian() {
return new MultivariateMatrixFunction() {
private static final long serialVersionUID = -2435076097232923678L;
public double[][] value(double[] point) {
return jacobian(point);
}
};
}
public abstract double[][] jacobian(double[] variables);
public abstract double[] value(double[] variables);
}
private static class LinearFullRankFunction extends MinpackFunction {
private static final long serialVersionUID = -9030323226268039536L;
public LinearFullRankFunction(int m, int n, double x0,
double theoreticalStartCost,
double theoreticalMinCost) {
super(m, buildArray(n, x0), theoreticalMinCost,
buildArray(n, -1.0));
}
@Override
public double[][] jacobian(double[] variables) {
double t = 2.0 / m;
double[][] jacobian = new double[m][];
for (int i = 0; i < m; ++i) {
jacobian[i] = new double[n];
for (int j = 0; j < n; ++j) {
jacobian[i][j] = (i == j) ? (1 - t) : -t;
}
}
return jacobian;
}
@Override
public double[] value(double[] variables) {
double sum = 0;
for (int i = 0; i < n; ++i) {
sum += variables[i];
}
double t = 1 + 2 * sum / m;
double[] f = new double[m];
for (int i = 0; i < n; ++i) {
f[i] = variables[i] - t;
}
Arrays.fill(f, n, m, -t);
return f;
}
}
private static class LinearRank1Function extends MinpackFunction {
private static final long serialVersionUID = 8494863245104608300L;
public LinearRank1Function(int m, int n, double x0,
double theoreticalStartCost,
double theoreticalMinCost) {
super(m, buildArray(n, x0), theoreticalMinCost, null);
}
@Override
public double[][] jacobian(double[] variables) {
double[][] jacobian = new double[m][];
for (int i = 0; i < m; ++i) {
jacobian[i] = new double[n];
for (int j = 0; j < n; ++j) {
jacobian[i][j] = (i + 1) * (j + 1);
}
}
return jacobian;
}
@Override
public double[] value(double[] variables) {
double[] f = new double[m];
double sum = 0;
for (int i = 0; i < n; ++i) {
sum += (i + 1) * variables[i];
}
for (int i = 0; i < m; ++i) {
f[i] = (i + 1) * sum - 1;
}
return f;
}
}
private static class LinearRank1ZeroColsAndRowsFunction extends MinpackFunction {
private static final long serialVersionUID = -3316653043091995018L;
public LinearRank1ZeroColsAndRowsFunction(int m, int n, double x0) {
super(m, buildArray(n, x0),
Math.sqrt((m * (m + 3) - 6) / (2.0 * (2 * m - 3))),
null);
}
@Override
public double[][] jacobian(double[] variables) {
double[][] jacobian = new double[m][];
for (int i = 0; i < m; ++i) {
jacobian[i] = new double[n];
jacobian[i][0] = 0;
for (int j = 1; j < (n - 1); ++j) {
if (i == 0) {
jacobian[i][j] = 0;
} else if (i != (m - 1)) {
jacobian[i][j] = i * (j + 1);
} else {
jacobian[i][j] = 0;
}
}
jacobian[i][n - 1] = 0;
}
return jacobian;
}
@Override
public double[] value(double[] variables) {
double[] f = new double[m];
double sum = 0;
for (int i = 1; i < (n - 1); ++i) {
sum += (i + 1) * variables[i];
}
for (int i = 0; i < (m - 1); ++i) {
f[i] = i * sum - 1;
}
f[m - 1] = -1;
return f;
}
}
private static class RosenbrockFunction extends MinpackFunction {
private static final long serialVersionUID = 2893438180956569134L;
public RosenbrockFunction(double[] startParams, double theoreticalStartCost) {
super(2, startParams, 0.0, buildArray(2, 1.0));
}
@Override
public double[][] jacobian(double[] variables) {
double x1 = variables[0];
return new double[][] { { -20 * x1, 10 }, { -1, 0 } };
}
@Override
public double[] value(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
return new double[] { 10 * (x2 - x1 * x1), 1 - x1 };
}
}
private static class HelicalValleyFunction extends MinpackFunction {
private static final long serialVersionUID = 220613787843200102L;
public HelicalValleyFunction(double[] startParams,
double theoreticalStartCost) {
super(3, startParams, 0.0, new double[] { 1.0, 0.0, 0.0 });
}
@Override
public double[][] jacobian(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
double tmpSquare = x1 * x1 + x2 * x2;
double tmp1 = twoPi * tmpSquare;
double tmp2 = Math.sqrt(tmpSquare);
return new double[][] {
{ 100 * x2 / tmp1, -100 * x1 / tmp1, 10 },
{ 10 * x1 / tmp2, 10 * x2 / tmp2, 0 },
{ 0, 0, 1 }
};
}
@Override
public double[] value(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
double x3 = variables[2];
double tmp1;
if (x1 == 0) {
tmp1 = (x2 >= 0) ? 0.25 : -0.25;
} else {
tmp1 = Math.atan(x2 / x1) / twoPi;
if (x1 < 0) {
tmp1 += 0.5;
}
}
double tmp2 = Math.sqrt(x1 * x1 + x2 * x2);
return new double[] {
10.0 * (x3 - 10 * tmp1),
10.0 * (tmp2 - 1),
x3
};
}
private static final double twoPi = 2.0 * Math.PI;
}
private static class PowellSingularFunction extends MinpackFunction {
private static final long serialVersionUID = 7298364171208142405L;
public PowellSingularFunction(double[] startParams,
double theoreticalStartCost) {
super(4, startParams, 0.0, buildArray(4, 0.0));
}
@Override
public double[][] jacobian(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
double x3 = variables[2];
double x4 = variables[3];
return new double[][] {
{ 1, 10, 0, 0 },
{ 0, 0, sqrt5, -sqrt5 },
{ 0, 2 * (x2 - 2 * x3), -4 * (x2 - 2 * x3), 0 },
{ 2 * sqrt10 * (x1 - x4), 0, 0, -2 * sqrt10 * (x1 - x4) }
};
}
@Override
public double[] value(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
double x3 = variables[2];
double x4 = variables[3];
return new double[] {
x1 + 10 * x2,
sqrt5 * (x3 - x4),
(x2 - 2 * x3) * (x2 - 2 * x3),
sqrt10 * (x1 - x4) * (x1 - x4)
};
}
private static final double sqrt5 = Math.sqrt( 5.0);
private static final double sqrt10 = Math.sqrt(10.0);
}
private static class FreudensteinRothFunction extends MinpackFunction {
private static final long serialVersionUID = 2892404999344244214L;
public FreudensteinRothFunction(double[] startParams,
double theoreticalStartCost,
double theoreticalMinCost,
double[] theoreticalMinParams) {
super(2, startParams, theoreticalMinCost,
theoreticalMinParams);
}
@Override
public double[][] jacobian(double[] variables) {
double x2 = variables[1];
return new double[][] {
{ 1, x2 * (10 - 3 * x2) - 2 },
{ 1, x2 * ( 2 + 3 * x2) - 14, }
};
}
@Override
public double[] value(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
return new double[] {
-13.0 + x1 + ((5.0 - x2) * x2 - 2.0) * x2,
-29.0 + x1 + ((1.0 + x2) * x2 - 14.0) * x2
};
}
}
private static class BardFunction extends MinpackFunction {
private static final long serialVersionUID = 5990442612572087668L;
public BardFunction(double x0,
double theoreticalStartCost,
double theoreticalMinCost,
double[] theoreticalMinParams) {
super(15, buildArray(3, x0), theoreticalMinCost,
theoreticalMinParams);
}
@Override
public double[][] jacobian(double[] variables) {
double x2 = variables[1];
double x3 = variables[2];
double[][] jacobian = new double[m][];
for (int i = 0; i < m; ++i) {
double tmp1 = i + 1;
double tmp2 = 15 - i;
double tmp3 = (i <= 7) ? tmp1 : tmp2;
double tmp4 = x2 * tmp2 + x3 * tmp3;
tmp4 *= tmp4;
jacobian[i] = new double[] { -1, tmp1 * tmp2 / tmp4, tmp1 * tmp3 / tmp4 };
}
return jacobian;
}
@Override
public double[] value(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
double x3 = variables[2];
double[] f = new double[m];
for (int i = 0; i < m; ++i) {
double tmp1 = i + 1;
double tmp2 = 15 - i;
double tmp3 = (i <= 7) ? tmp1 : tmp2;
f[i] = y[i] - (x1 + tmp1 / (x2 * tmp2 + x3 * tmp3));
}
return f;
}
private static final double[] y = {
0.14, 0.18, 0.22, 0.25, 0.29,
0.32, 0.35, 0.39, 0.37, 0.58,
0.73, 0.96, 1.34, 2.10, 4.39
};
}
private static class KowalikOsborneFunction extends MinpackFunction {
private static final long serialVersionUID = -4867445739880495801L;
public KowalikOsborneFunction(double[] startParams,
double theoreticalStartCost,
double theoreticalMinCost,
double[] theoreticalMinParams) {
super(11, startParams, theoreticalMinCost,
theoreticalMinParams);
if (theoreticalStartCost > 20.0) {
setCostAccuracy(2.0e-4);
setParamsAccuracy(5.0e-3);
}
}
@Override
public double[][] jacobian(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
double x3 = variables[2];
double x4 = variables[3];
double[][] jacobian = new double[m][];
for (int i = 0; i < m; ++i) {
double tmp = v[i] * (v[i] + x3) + x4;
double j1 = -v[i] * (v[i] + x2) / tmp;
double j2 = -v[i] * x1 / tmp;
double j3 = j1 * j2;
double j4 = j3 / v[i];
jacobian[i] = new double[] { j1, j2, j3, j4 };
}
return jacobian;
}
@Override
public double[] value(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
double x3 = variables[2];
double x4 = variables[3];
double[] f = new double[m];
for (int i = 0; i < m; ++i) {
f[i] = y[i] - x1 * (v[i] * (v[i] + x2)) / (v[i] * (v[i] + x3) + x4);
}
return f;
}
private static final double[] v = {
4.0, 2.0, 1.0, 0.5, 0.25, 0.167, 0.125, 0.1, 0.0833, 0.0714, 0.0625
};
private static final double[] y = {
0.1957, 0.1947, 0.1735, 0.1600, 0.0844, 0.0627,
0.0456, 0.0342, 0.0323, 0.0235, 0.0246
};
}
private static class MeyerFunction extends MinpackFunction {
private static final long serialVersionUID = -838060619150131027L;
public MeyerFunction(double[] startParams,
double theoreticalStartCost,
double theoreticalMinCost,
double[] theoreticalMinParams) {
super(16, startParams, theoreticalMinCost,
theoreticalMinParams);
if (theoreticalStartCost > 1.0e6) {
setCostAccuracy(7.0e-3);
setParamsAccuracy(2.0e-2);
}
}
@Override
public double[][] jacobian(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
double x3 = variables[2];
double[][] jacobian = new double[m][];
for (int i = 0; i < m; ++i) {
double temp = 5.0 * (i + 1) + 45.0 + x3;
double tmp1 = x2 / temp;
double tmp2 = Math.exp(tmp1);
double tmp3 = x1 * tmp2 / temp;
jacobian[i] = new double[] { tmp2, tmp3, -tmp1 * tmp3 };
}
return jacobian;
}
@Override
public double[] value(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
double x3 = variables[2];
double[] f = new double[m];
for (int i = 0; i < m; ++i) {
f[i] = x1 * Math.exp(x2 / (5.0 * (i + 1) + 45.0 + x3)) - y[i];
}
return f;
}
private static final double[] y = {
34780.0, 28610.0, 23650.0, 19630.0,
16370.0, 13720.0, 11540.0, 9744.0,
8261.0, 7030.0, 6005.0, 5147.0,
4427.0, 3820.0, 3307.0, 2872.0
};
}
private static class WatsonFunction extends MinpackFunction {
private static final long serialVersionUID = -9034759294980218927L;
public WatsonFunction(int n, double x0,
double theoreticalStartCost,
double theoreticalMinCost,
double[] theoreticalMinParams) {
super(31, buildArray(n, x0), theoreticalMinCost,
theoreticalMinParams);
}
@Override
public double[][] jacobian(double[] variables) {
double[][] jacobian = new double[m][];
for (int i = 0; i < (m - 2); ++i) {
double div = (i + 1) / 29.0;
double s2 = 0.0;
double dx = 1.0;
for (int j = 0; j < n; ++j) {
s2 += dx * variables[j];
dx *= div;
}
double temp= 2 * div * s2;
dx = 1.0 / div;
jacobian[i] = new double[n];
for (int j = 0; j < n; ++j) {
jacobian[i][j] = dx * (j - temp);
dx *= div;
}
}
jacobian[m - 2] = new double[n];
jacobian[m - 2][0] = 1;
jacobian[m - 1] = new double[n];
jacobian[m - 1][0]= -2 * variables[0];
jacobian[m - 1][1]= 1;
return jacobian;
}
@Override
public double[] value(double[] variables) {
double[] f = new double[m];
for (int i = 0; i < (m - 2); ++i) {
double div = (i + 1) / 29.0;
double s1 = 0;
double dx = 1;
for (int j = 1; j < n; ++j) {
s1 += j * dx * variables[j];
dx *= div;
}
double s2 =0;
dx =1;
for (int j = 0; j < n; ++j) {
s2 += dx * variables[j];
dx *= div;
}
f[i] = s1 - s2 * s2 - 1;
}
double x1 = variables[0];
double x2 = variables[1];
f[m - 2] = x1;
f[m - 1] = x2 - x1 * x1 - 1;
return f;
}
}
private static class Box3DimensionalFunction extends MinpackFunction {
private static final long serialVersionUID = 5511403858142574493L;
public Box3DimensionalFunction(int m, double[] startParams,
double theoreticalStartCost) {
super(m, startParams, 0.0,
new double[] { 1.0, 10.0, 1.0 });
}
@Override
public double[][] jacobian(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
double[][] jacobian = new double[m][];
for (int i = 0; i < m; ++i) {
double tmp = (i + 1) / 10.0;
jacobian[i] = new double[] {
-tmp * Math.exp(-tmp * x1),
tmp * Math.exp(-tmp * x2),
Math.exp(-i - 1) - Math.exp(-tmp)
};
}
return jacobian;
}
@Override
public double[] value(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
double x3 = variables[2];
double[] f = new double[m];
for (int i = 0; i < m; ++i) {
double tmp = (i + 1) / 10.0;
f[i] = Math.exp(-tmp * x1) - Math.exp(-tmp * x2)
+ (Math.exp(-i - 1) - Math.exp(-tmp)) * x3;
}
return f;
}
}
private static class JennrichSampsonFunction extends MinpackFunction {
private static final long serialVersionUID = -2489165190443352947L;
public JennrichSampsonFunction(int m, double[] startParams,
double theoreticalStartCost,
double theoreticalMinCost,
double[] theoreticalMinParams) {
super(m, startParams, theoreticalMinCost,
theoreticalMinParams);
}
@Override
public double[][] jacobian(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
double[][] jacobian = new double[m][];
for (int i = 0; i < m; ++i) {
double t = i + 1;
jacobian[i] = new double[] { -t * Math.exp(t * x1), -t * Math.exp(t * x2) };
}
return jacobian;
}
@Override
public double[] value(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
double[] f = new double[m];
for (int i = 0; i < m; ++i) {
double temp = i + 1;
f[i] = 2 + 2 * temp - Math.exp(temp * x1) - Math.exp(temp * x2);
}
return f;
}
}
private static class BrownDennisFunction extends MinpackFunction {
private static final long serialVersionUID = 8340018645694243910L;
public BrownDennisFunction(int m, double[] startParams,
double theoreticalStartCost,
double theoreticalMinCost,
double[] theoreticalMinParams) {
super(m, startParams, theoreticalMinCost,
theoreticalMinParams);
setCostAccuracy(2.5e-8);
}
@Override
public double[][] jacobian(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
double x3 = variables[2];
double x4 = variables[3];
double[][] jacobian = new double[m][];
for (int i = 0; i < m; ++i) {
double temp = (i + 1) / 5.0;
double ti = Math.sin(temp);
double tmp1 = x1 + temp * x2 - Math.exp(temp);
double tmp2 = x3 + ti * x4 - Math.cos(temp);
jacobian[i] = new double[] {
2 * tmp1, 2 * temp * tmp1, 2 * tmp2, 2 * ti * tmp2
};
}
return jacobian;
}
@Override
public double[] value(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
double x3 = variables[2];
double x4 = variables[3];
double[] f = new double[m];
for (int i = 0; i < m; ++i) {
double temp = (i + 1) / 5.0;
double tmp1 = x1 + temp * x2 - Math.exp(temp);
double tmp2 = x3 + Math.sin(temp) * x4 - Math.cos(temp);
f[i] = tmp1 * tmp1 + tmp2 * tmp2;
}
return f;
}
}
private static class ChebyquadFunction extends MinpackFunction {
private static final long serialVersionUID = -2394877275028008594L;
private static double[] buildChebyquadArray(int n, double factor) {
double[] array = new double[n];
double inv = factor / (n + 1);
for (int i = 0; i < n; ++i) {
array[i] = (i + 1) * inv;
}
return array;
}
public ChebyquadFunction(int n, int m, double factor,
double theoreticalStartCost,
double theoreticalMinCost,
double[] theoreticalMinParams) {
super(m, buildChebyquadArray(n, factor), theoreticalMinCost,
theoreticalMinParams);
}
@Override
public double[][] jacobian(double[] variables) {
double[][] jacobian = new double[m][];
for (int i = 0; i < m; ++i) {
jacobian[i] = new double[n];
}
double dx = 1.0 / n;
for (int j = 0; j < n; ++j) {
double tmp1 = 1;
double tmp2 = 2 * variables[j] - 1;
double temp = 2 * tmp2;
double tmp3 = 0;
double tmp4 = 2;
for (int i = 0; i < m; ++i) {
jacobian[i][j] = dx * tmp4;
double ti = 4 * tmp2 + temp * tmp4 - tmp3;
tmp3 = tmp4;
tmp4 = ti;
ti = temp * tmp2 - tmp1;
tmp1 = tmp2;
tmp2 = ti;
}
}
return jacobian;
}
@Override
public double[] value(double[] variables) {
double[] f = new double[m];
for (int j = 0; j < n; ++j) {
double tmp1 = 1;
double tmp2 = 2 * variables[j] - 1;
double temp = 2 * tmp2;
for (int i = 0; i < m; ++i) {
f[i] += tmp2;
double ti = temp * tmp2 - tmp1;
tmp1 = tmp2;
tmp2 = ti;
}
}
double dx = 1.0 / n;
boolean iev = false;
for (int i = 0; i < m; ++i) {
f[i] *= dx;
if (iev) {
f[i] += 1.0 / (i * (i + 2));
}
iev = ! iev;
}
return f;
}
}
private static class BrownAlmostLinearFunction extends MinpackFunction {
private static final long serialVersionUID = 8239594490466964725L;
public BrownAlmostLinearFunction(int m, double factor,
double theoreticalStartCost,
double theoreticalMinCost,
double[] theoreticalMinParams) {
super(m, buildArray(m, factor), theoreticalMinCost,
theoreticalMinParams);
}
@Override
public double[][] jacobian(double[] variables) {
double[][] jacobian = new double[m][];
for (int i = 0; i < m; ++i) {
jacobian[i] = new double[n];
}
double prod = 1;
for (int j = 0; j < n; ++j) {
prod *= variables[j];
for (int i = 0; i < n; ++i) {
jacobian[i][j] = 1;
}
jacobian[j][j] = 2;
}
for (int j = 0; j < n; ++j) {
double temp = variables[j];
if (temp == 0) {
temp = 1;
prod = 1;
for (int k = 0; k < n; ++k) {
if (k != j) {
prod *= variables[k];
}
}
}
jacobian[n - 1][j] = prod / temp;
}
return jacobian;
}
@Override
public double[] value(double[] variables) {
double[] f = new double[m];
double sum = -(n + 1);
double prod = 1;
for (int j = 0; j < n; ++j) {
sum += variables[j];
prod *= variables[j];
}
for (int i = 0; i < n; ++i) {
f[i] = variables[i] + sum;
}
f[n - 1] = prod - 1;
return f;
}
}
private static class Osborne1Function extends MinpackFunction {
private static final long serialVersionUID = 4006743521149849494L;
public Osborne1Function(double[] startParams,
double theoreticalStartCost,
double theoreticalMinCost,
double[] theoreticalMinParams) {
super(33, startParams, theoreticalMinCost,
theoreticalMinParams);
}
@Override
public double[][] jacobian(double[] variables) {
double x2 = variables[1];
double x3 = variables[2];
double x4 = variables[3];
double x5 = variables[4];
double[][] jacobian = new double[m][];
for (int i = 0; i < m; ++i) {
double temp = 10.0 * i;
double tmp1 = Math.exp(-temp * x4);
double tmp2 = Math.exp(-temp * x5);
jacobian[i] = new double[] {
-1, -tmp1, -tmp2, temp * x2 * tmp1, temp * x3 * tmp2
};
}
return jacobian;
}
@Override
public double[] value(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
double x3 = variables[2];
double x4 = variables[3];
double x5 = variables[4];
double[] f = new double[m];
for (int i = 0; i < m; ++i) {
double temp = 10.0 * i;
double tmp1 = Math.exp(-temp * x4);
double tmp2 = Math.exp(-temp * x5);
f[i] = y[i] - (x1 + x2 * tmp1 + x3 * tmp2);
}
return f;
}
private static final double[] y = {
0.844, 0.908, 0.932, 0.936, 0.925, 0.908, 0.881, 0.850, 0.818, 0.784, 0.751,
0.718, 0.685, 0.658, 0.628, 0.603, 0.580, 0.558, 0.538, 0.522, 0.506, 0.490,
0.478, 0.467, 0.457, 0.448, 0.438, 0.431, 0.424, 0.420, 0.414, 0.411, 0.406
};
}
private static class Osborne2Function extends MinpackFunction {
private static final long serialVersionUID = -8418268780389858746L;
public Osborne2Function(double[] startParams,
double theoreticalStartCost,
double theoreticalMinCost,
double[] theoreticalMinParams) {
super(65, startParams, theoreticalMinCost,
theoreticalMinParams);
}
@Override
public double[][] jacobian(double[] variables) {
double x01 = variables[0];
double x02 = variables[1];
double x03 = variables[2];
double x04 = variables[3];
double x05 = variables[4];
double x06 = variables[5];
double x07 = variables[6];
double x08 = variables[7];
double x09 = variables[8];
double x10 = variables[9];
double x11 = variables[10];
double[][] jacobian = new double[m][];
for (int i = 0; i < m; ++i) {
double temp = i / 10.0;
double tmp1 = Math.exp(-x05 * temp);
double tmp2 = Math.exp(-x06 * (temp - x09) * (temp - x09));
double tmp3 = Math.exp(-x07 * (temp - x10) * (temp - x10));
double tmp4 = Math.exp(-x08 * (temp - x11) * (temp - x11));
jacobian[i] = new double[] {
-tmp1,
-tmp2,
-tmp3,
-tmp4,
temp * x01 * tmp1,
x02 * (temp - x09) * (temp - x09) * tmp2,
x03 * (temp - x10) * (temp - x10) * tmp3,
x04 * (temp - x11) * (temp - x11) * tmp4,
-2 * x02 * x06 * (temp - x09) * tmp2,
-2 * x03 * x07 * (temp - x10) * tmp3,
-2 * x04 * x08 * (temp - x11) * tmp4
};
}
return jacobian;
}
@Override
public double[] value(double[] variables) {
double x01 = variables[0];
double x02 = variables[1];
double x03 = variables[2];
double x04 = variables[3];
double x05 = variables[4];
double x06 = variables[5];
double x07 = variables[6];
double x08 = variables[7];
double x09 = variables[8];
double x10 = variables[9];
double x11 = variables[10];
double[] f = new double[m];
for (int i = 0; i < m; ++i) {
double temp = i / 10.0;
double tmp1 = Math.exp(-x05 * temp);
double tmp2 = Math.exp(-x06 * (temp - x09) * (temp - x09));
double tmp3 = Math.exp(-x07 * (temp - x10) * (temp - x10));
double tmp4 = Math.exp(-x08 * (temp - x11) * (temp - x11));
f[i] = y[i] - (x01 * tmp1 + x02 * tmp2 + x03 * tmp3 + x04 * tmp4);
}
return f;
}
private static final double[] y = {
1.366, 1.191, 1.112, 1.013, 0.991,
0.885, 0.831, 0.847, 0.786, 0.725,
0.746, 0.679, 0.608, 0.655, 0.616,
0.606, 0.602, 0.626, 0.651, 0.724,
0.649, 0.649, 0.694, 0.644, 0.624,
0.661, 0.612, 0.558, 0.533, 0.495,
0.500, 0.423, 0.395, 0.375, 0.372,
0.391, 0.396, 0.405, 0.428, 0.429,
0.523, 0.562, 0.607, 0.653, 0.672,
0.708, 0.633, 0.668, 0.645, 0.632,
0.591, 0.559, 0.597, 0.625, 0.739,
0.710, 0.729, 0.720, 0.636, 0.581,
0.428, 0.292, 0.162, 0.098, 0.054
};
}
} | // You are a professional Java test case writer, please create a test case named `testMinpackJennrichSampson` for the issue `Math-MATH-405`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Math-MATH-405
//
// ## Issue-Title:
// Inconsistent result from Levenberg-Marquardt
//
// ## Issue-Description:
//
// Levenberg-Marquardt (its method doOptimize) returns a VectorialPointValuePair. However, the class holds the optimum point, the vector of the objective function, the cost and residuals. The value returns by doOptimize does not always corresponds to the point which leads to the residuals and cost
//
//
//
//
//
public void testMinpackJennrichSampson() {
| 330 | 64 | 323 | src/test/java/org/apache/commons/math/optimization/general/MinpackTest.java | src/test/java | ```markdown
## Issue-ID: Math-MATH-405
## Issue-Title:
Inconsistent result from Levenberg-Marquardt
## Issue-Description:
Levenberg-Marquardt (its method doOptimize) returns a VectorialPointValuePair. However, the class holds the optimum point, the vector of the objective function, the cost and residuals. The value returns by doOptimize does not always corresponds to the point which leads to the residuals and cost
```
You are a professional Java test case writer, please create a test case named `testMinpackJennrichSampson` for the issue `Math-MATH-405`, utilizing the provided issue report information and the following function signature.
```java
public void testMinpackJennrichSampson() {
```
| 323 | [
"org.apache.commons.math.optimization.general.LevenbergMarquardtOptimizer"
] | 7f53fd8c031b37c1f534faeca6fa8b57509dc69909fdf3a6593613a0de61bc94 | public void testMinpackJennrichSampson() | // You are a professional Java test case writer, please create a test case named `testMinpackJennrichSampson` for the issue `Math-MATH-405`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Math-MATH-405
//
// ## Issue-Title:
// Inconsistent result from Levenberg-Marquardt
//
// ## Issue-Description:
//
// Levenberg-Marquardt (its method doOptimize) returns a VectorialPointValuePair. However, the class holds the optimum point, the vector of the objective function, the cost and residuals. The value returns by doOptimize does not always corresponds to the point which leads to the residuals and cost
//
//
//
//
//
| Math | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.math.optimization.general;
import java.io.Serializable;
import java.util.Arrays;
import junit.framework.TestCase;
import org.apache.commons.math.FunctionEvaluationException;
import org.apache.commons.math.analysis.DifferentiableMultivariateVectorialFunction;
import org.apache.commons.math.analysis.MultivariateMatrixFunction;
import org.apache.commons.math.optimization.OptimizationException;
import org.apache.commons.math.optimization.VectorialPointValuePair;
/**
* <p>Some of the unit tests are re-implementations of the MINPACK <a
* href="http://www.netlib.org/minpack/ex/file17">file17</a> and <a
* href="http://www.netlib.org/minpack/ex/file22">file22</a> test files.
* The redistribution policy for MINPACK is available <a
* href="http://www.netlib.org/minpack/disclaimer">here</a>, for
* convenience, it is reproduced below.</p>
* <table border="0" width="80%" cellpadding="10" align="center" bgcolor="#E0E0E0">
* <tr><td>
* Minpack Copyright Notice (1999) University of Chicago.
* All rights reserved
* </td></tr>
* <tr><td>
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
* <ol>
* <li>Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.</li>
* <li>Redistributions in binary form must reproduce the above
* copyright notice, this list of conditions and the following
* disclaimer in the documentation and/or other materials provided
* with the distribution.</li>
* <li>The end-user documentation included with the redistribution, if any,
* must include the following acknowledgment:
* <code>This product includes software developed by the University of
* Chicago, as Operator of Argonne National Laboratory.</code>
* Alternately, this acknowledgment may appear in the software itself,
* if and wherever such third-party acknowledgments normally appear.</li>
* <li><strong>WARRANTY DISCLAIMER. THE SOFTWARE IS SUPPLIED "AS IS"
* WITHOUT WARRANTY OF ANY KIND. THE COPYRIGHT HOLDER, THE
* UNITED STATES, THE UNITED STATES DEPARTMENT OF ENERGY, AND
* THEIR EMPLOYEES: (1) DISCLAIM ANY WARRANTIES, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO ANY IMPLIED WARRANTIES
* OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE, TITLE
* OR NON-INFRINGEMENT, (2) DO NOT ASSUME ANY LEGAL LIABILITY
* OR RESPONSIBILITY FOR THE ACCURACY, COMPLETENESS, OR
* USEFULNESS OF THE SOFTWARE, (3) DO NOT REPRESENT THAT USE OF
* THE SOFTWARE WOULD NOT INFRINGE PRIVATELY OWNED RIGHTS, (4)
* DO NOT WARRANT THAT THE SOFTWARE WILL FUNCTION
* UNINTERRUPTED, THAT IT IS ERROR-FREE OR THAT ANY ERRORS WILL
* BE CORRECTED.</strong></li>
* <li><strong>LIMITATION OF LIABILITY. IN NO EVENT WILL THE COPYRIGHT
* HOLDER, THE UNITED STATES, THE UNITED STATES DEPARTMENT OF
* ENERGY, OR THEIR EMPLOYEES: BE LIABLE FOR ANY INDIRECT,
* INCIDENTAL, CONSEQUENTIAL, SPECIAL OR PUNITIVE DAMAGES OF
* ANY KIND OR NATURE, INCLUDING BUT NOT LIMITED TO LOSS OF
* PROFITS OR LOSS OF DATA, FOR ANY REASON WHATSOEVER, WHETHER
* SUCH LIABILITY IS ASSERTED ON THE BASIS OF CONTRACT, TORT
* (INCLUDING NEGLIGENCE OR STRICT LIABILITY), OR OTHERWISE,
* EVEN IF ANY OF SAID PARTIES HAS BEEN WARNED OF THE
* POSSIBILITY OF SUCH LOSS OR DAMAGES.</strong></li>
* <ol></td></tr>
* </table>
* @author Argonne National Laboratory. MINPACK project. March 1980 (original fortran minpack tests)
* @author Burton S. Garbow (original fortran minpack tests)
* @author Kenneth E. Hillstrom (original fortran minpack tests)
* @author Jorge J. More (original fortran minpack tests)
* @author Luc Maisonobe (non-minpack tests and minpack tests Java translation)
*/
public class MinpackTest extends TestCase {
public MinpackTest(String name) {
super(name);
}
public void testMinpackLinearFullRank() {
minpackTest(new LinearFullRankFunction(10, 5, 1.0,
5.0, 2.23606797749979), false);
minpackTest(new LinearFullRankFunction(50, 5, 1.0,
8.06225774829855, 6.70820393249937), false);
}
public void testMinpackLinearRank1() {
minpackTest(new LinearRank1Function(10, 5, 1.0,
291.521868819476, 1.4638501094228), false);
minpackTest(new LinearRank1Function(50, 5, 1.0,
3101.60039334535, 3.48263016573496), false);
}
public void testMinpackLinearRank1ZeroColsAndRows() {
minpackTest(new LinearRank1ZeroColsAndRowsFunction(10, 5, 1.0), false);
minpackTest(new LinearRank1ZeroColsAndRowsFunction(50, 5, 1.0), false);
}
public void testMinpackRosenbrok() {
minpackTest(new RosenbrockFunction(new double[] { -1.2, 1.0 },
Math.sqrt(24.2)), false);
minpackTest(new RosenbrockFunction(new double[] { -12.0, 10.0 },
Math.sqrt(1795769.0)), false);
minpackTest(new RosenbrockFunction(new double[] { -120.0, 100.0 },
11.0 * Math.sqrt(169000121.0)), false);
}
public void testMinpackHelicalValley() {
minpackTest(new HelicalValleyFunction(new double[] { -1.0, 0.0, 0.0 },
50.0), false);
minpackTest(new HelicalValleyFunction(new double[] { -10.0, 0.0, 0.0 },
102.95630140987), false);
minpackTest(new HelicalValleyFunction(new double[] { -100.0, 0.0, 0.0},
991.261822123701), false);
}
public void testMinpackPowellSingular() {
minpackTest(new PowellSingularFunction(new double[] { 3.0, -1.0, 0.0, 1.0 },
14.6628782986152), false);
minpackTest(new PowellSingularFunction(new double[] { 30.0, -10.0, 0.0, 10.0 },
1270.9838708654), false);
minpackTest(new PowellSingularFunction(new double[] { 300.0, -100.0, 0.0, 100.0 },
126887.903284750), false);
}
public void testMinpackFreudensteinRoth() {
minpackTest(new FreudensteinRothFunction(new double[] { 0.5, -2.0 },
20.0124960961895, 6.99887517584575,
new double[] {
11.4124844654993,
-0.896827913731509
}), false);
minpackTest(new FreudensteinRothFunction(new double[] { 5.0, -20.0 },
12432.833948863, 6.9988751744895,
new double[] {
11.41300466147456,
-0.896796038685959
}), false);
minpackTest(new FreudensteinRothFunction(new double[] { 50.0, -200.0 },
11426454.595762, 6.99887517242903,
new double[] {
11.412781785788564,
-0.8968051074920405
}), false);
}
public void testMinpackBard() {
minpackTest(new BardFunction(1.0, 6.45613629515967, 0.0906359603390466,
new double[] {
0.0824105765758334,
1.1330366534715,
2.34369463894115
}), false);
minpackTest(new BardFunction(10.0, 36.1418531596785, 4.17476870138539,
new double[] {
0.840666673818329,
-158848033.259565,
-164378671.653535
}), false);
minpackTest(new BardFunction(100.0, 384.114678637399, 4.17476870135969,
new double[] {
0.840666673867645,
-158946167.205518,
-164464906.857771
}), false);
}
public void testMinpackKowalikOsborne() {
minpackTest(new KowalikOsborneFunction(new double[] { 0.25, 0.39, 0.415, 0.39 },
0.0728915102882945,
0.017535837721129,
new double[] {
0.192807810476249,
0.191262653354071,
0.123052801046931,
0.136053221150517
}), false);
minpackTest(new KowalikOsborneFunction(new double[] { 2.5, 3.9, 4.15, 3.9 },
2.97937007555202,
0.032052192917937,
new double[] {
728675.473768287,
-14.0758803129393,
-32977797.7841797,
-20571594.1977912
}), false);
minpackTest(new KowalikOsborneFunction(new double[] { 25.0, 39.0, 41.5, 39.0 },
29.9590617016037,
0.0175364017658228,
new double[] {
0.192948328597594,
0.188053165007911,
0.122430604321144,
0.134575665392506
}), false);
}
public void testMinpackMeyer() {
minpackTest(new MeyerFunction(new double[] { 0.02, 4000.0, 250.0 },
41153.4665543031, 9.37794514651874,
new double[] {
0.00560963647102661,
6181.34634628659,
345.223634624144
}), false);
minpackTest(new MeyerFunction(new double[] { 0.2, 40000.0, 2500.0 },
4168216.89130846, 792.917871779501,
new double[] {
1.42367074157994e-11,
33695.7133432541,
901.268527953801
}), true);
}
public void testMinpackWatson() {
minpackTest(new WatsonFunction(6, 0.0,
5.47722557505166, 0.0478295939097601,
new double[] {
-0.0157249615083782, 1.01243488232965,
-0.232991722387673, 1.26043101102818,
-1.51373031394421, 0.99299727291842
}), false);
minpackTest(new WatsonFunction(6, 10.0,
6433.12578950026, 0.0478295939096951,
new double[] {
-0.0157251901386677, 1.01243485860105,
-0.232991545843829, 1.26042932089163,
-1.51372776706575, 0.99299573426328
}), false);
minpackTest(new WatsonFunction(6, 100.0,
674256.040605213, 0.047829593911544,
new double[] {
-0.0157247019712586, 1.01243490925658,
-0.232991922761641, 1.26043292929555,
-1.51373320452707, 0.99299901922322
}), false);
minpackTest(new WatsonFunction(9, 0.0,
5.47722557505166, 0.00118311459212420,
new double[] {
-0.153070644166722e-4, 0.999789703934597,
0.0147639634910978, 0.146342330145992,
1.00082109454817, -2.61773112070507,
4.10440313943354, -3.14361226236241,
1.05262640378759
}), false);
minpackTest(new WatsonFunction(9, 10.0,
12088.127069307, 0.00118311459212513,
new double[] {
-0.153071334849279e-4, 0.999789703941234,
0.0147639629786217, 0.146342334818836,
1.00082107321386, -2.61773107084722,
4.10440307655564, -3.14361222178686,
1.05262639322589
}), false);
minpackTest(new WatsonFunction(9, 100.0,
1269109.29043834, 0.00118311459212384,
new double[] {
-0.153069523352176e-4, 0.999789703958371,
0.0147639625185392, 0.146342341096326,
1.00082104729164, -2.61773101573645,
4.10440301427286, -3.14361218602503,
1.05262638516774
}), false);
minpackTest(new WatsonFunction(12, 0.0,
5.47722557505166, 0.217310402535861e-4,
new double[] {
-0.660266001396382e-8, 1.00000164411833,
-0.000563932146980154, 0.347820540050756,
-0.156731500244233, 1.05281515825593,
-3.24727109519451, 7.2884347837505,
-10.271848098614, 9.07411353715783,
-4.54137541918194, 1.01201187975044
}), false);
minpackTest(new WatsonFunction(12, 10.0,
19220.7589790951, 0.217310402518509e-4,
new double[] {
-0.663710223017410e-8, 1.00000164411787,
-0.000563932208347327, 0.347820540486998,
-0.156731503955652, 1.05281517654573,
-3.2472711515214, 7.28843489430665,
-10.2718482369638, 9.07411364383733,
-4.54137546533666, 1.01201188830857
}), false);
minpackTest(new WatsonFunction(12, 100.0,
2018918.04462367, 0.217310402539845e-4,
new double[] {
-0.663806046485249e-8, 1.00000164411786,
-0.000563932210324959, 0.347820540503588,
-0.156731504091375, 1.05281517718031,
-3.24727115337025, 7.28843489775302,
-10.2718482410813, 9.07411364688464,
-4.54137546660822, 1.0120118885369
}), false);
}
public void testMinpackBox3Dimensional() {
minpackTest(new Box3DimensionalFunction(10, new double[] { 0.0, 10.0, 20.0 },
32.1115837449572), false);
}
public void testMinpackJennrichSampson() {
minpackTest(new JennrichSampsonFunction(10, new double[] { 0.3, 0.4 },
64.5856498144943, 11.1517793413499,
new double[] {
// 0.2578330049, 0.257829976764542
0.2578199266368004, 0.25782997676455244
}), false);
}
public void testMinpackBrownDennis() {
minpackTest(new BrownDennisFunction(20,
new double[] { 25.0, 5.0, -5.0, -1.0 },
2815.43839161816, 292.954288244866,
new double[] {
-11.59125141003, 13.2024883984741,
-0.403574643314272, 0.236736269844604
}), false);
minpackTest(new BrownDennisFunction(20,
new double[] { 250.0, 50.0, -50.0, -10.0 },
555073.354173069, 292.954270581415,
new double[] {
-11.5959274272203, 13.2041866926242,
-0.403417362841545, 0.236771143410386
}), false);
minpackTest(new BrownDennisFunction(20,
new double[] { 2500.0, 500.0, -500.0, -100.0 },
61211252.2338581, 292.954306151134,
new double[] {
-11.5902596937374, 13.2020628854665,
-0.403688070279258, 0.236665033746463
}), false);
}
public void testMinpackChebyquad() {
minpackTest(new ChebyquadFunction(1, 8, 1.0,
1.88623796907732, 1.88623796907732,
new double[] { 0.5 }), false);
minpackTest(new ChebyquadFunction(1, 8, 10.0,
5383344372.34005, 1.88424820499951,
new double[] { 0.9817314924684 }), false);
minpackTest(new ChebyquadFunction(1, 8, 100.0,
0.118088726698392e19, 1.88424820499347,
new double[] { 0.9817314852934 }), false);
minpackTest(new ChebyquadFunction(8, 8, 1.0,
0.196513862833975, 0.0593032355046727,
new double[] {
0.0431536648587336, 0.193091637843267,
0.266328593812698, 0.499999334628884,
0.500000665371116, 0.733671406187302,
0.806908362156733, 0.956846335141266
}), false);
minpackTest(new ChebyquadFunction(9, 9, 1.0,
0.16994993465202, 0.0,
new double[] {
0.0442053461357828, 0.199490672309881,
0.23561910847106, 0.416046907892598,
0.5, 0.583953092107402,
0.764380891528940, 0.800509327690119,
0.955794653864217
}), false);
minpackTest(new ChebyquadFunction(10, 10, 1.0,
0.183747831178711, 0.0806471004038253,
new double[] {
0.0596202671753563, 0.166708783805937,
0.239171018813509, 0.398885290346268,
0.398883667870681, 0.601116332129320,
0.60111470965373, 0.760828981186491,
0.833291216194063, 0.940379732824644
}), false);
}
public void testMinpackBrownAlmostLinear() {
minpackTest(new BrownAlmostLinearFunction(10, 0.5,
16.5302162063499, 0.0,
new double[] {
0.979430303349862, 0.979430303349862,
0.979430303349862, 0.979430303349862,
0.979430303349862, 0.979430303349862,
0.979430303349862, 0.979430303349862,
0.979430303349862, 1.20569696650138
}), false);
minpackTest(new BrownAlmostLinearFunction(10, 5.0,
9765624.00089211, 0.0,
new double[] {
0.979430303349865, 0.979430303349865,
0.979430303349865, 0.979430303349865,
0.979430303349865, 0.979430303349865,
0.979430303349865, 0.979430303349865,
0.979430303349865, 1.20569696650135
}), false);
minpackTest(new BrownAlmostLinearFunction(10, 50.0,
0.9765625e17, 0.0,
new double[] {
1.0, 1.0, 1.0, 1.0, 1.0,
1.0, 1.0, 1.0, 1.0, 1.0
}), false);
minpackTest(new BrownAlmostLinearFunction(30, 0.5,
83.476044467848, 0.0,
new double[] {
0.997754216442807, 0.997754216442807,
0.997754216442807, 0.997754216442807,
0.997754216442807, 0.997754216442807,
0.997754216442807, 0.997754216442807,
0.997754216442807, 0.997754216442807,
0.997754216442807, 0.997754216442807,
0.997754216442807, 0.997754216442807,
0.997754216442807, 0.997754216442807,
0.997754216442807, 0.997754216442807,
0.997754216442807, 0.997754216442807,
0.997754216442807, 0.997754216442807,
0.997754216442807, 0.997754216442807,
0.997754216442807, 0.997754216442807,
0.997754216442807, 0.997754216442807,
0.997754216442807, 1.06737350671578
}), false);
minpackTest(new BrownAlmostLinearFunction(40, 0.5,
128.026364472323, 0.0,
new double[] {
1.00000000000002, 1.00000000000002,
1.00000000000002, 1.00000000000002,
1.00000000000002, 1.00000000000002,
1.00000000000002, 1.00000000000002,
1.00000000000002, 1.00000000000002,
1.00000000000002, 1.00000000000002,
1.00000000000002, 1.00000000000002,
1.00000000000002, 1.00000000000002,
1.00000000000002, 1.00000000000002,
1.00000000000002, 1.00000000000002,
1.00000000000002, 1.00000000000002,
1.00000000000002, 1.00000000000002,
1.00000000000002, 1.00000000000002,
1.00000000000002, 1.00000000000002,
1.00000000000002, 1.00000000000002,
1.00000000000002, 1.00000000000002,
1.00000000000002, 1.00000000000002,
0.999999999999121
}), false);
}
public void testMinpackOsborne1() {
minpackTest(new Osborne1Function(new double[] { 0.5, 1.5, -1.0, 0.01, 0.02, },
0.937564021037838, 0.00739249260904843,
new double[] {
0.375410049244025, 1.93584654543108,
-1.46468676748716, 0.0128675339110439,
0.0221227011813076
}), false);
}
public void testMinpackOsborne2() {
minpackTest(new Osborne2Function(new double[] {
1.3, 0.65, 0.65, 0.7, 0.6,
3.0, 5.0, 7.0, 2.0, 4.5, 5.5
},
1.44686540984712, 0.20034404483314,
new double[] {
1.30997663810096, 0.43155248076,
0.633661261602859, 0.599428560991695,
0.754179768272449, 0.904300082378518,
1.36579949521007, 4.82373199748107,
2.39868475104871, 4.56887554791452,
5.67534206273052
}), false);
}
private void minpackTest(MinpackFunction function, boolean exceptionExpected) {
LevenbergMarquardtOptimizer optimizer = new LevenbergMarquardtOptimizer();
optimizer.setMaxIterations(100 * (function.getN() + 1));
optimizer.setCostRelativeTolerance(Math.sqrt(2.22044604926e-16));
optimizer.setParRelativeTolerance(Math.sqrt(2.22044604926e-16));
optimizer.setOrthoTolerance(2.22044604926e-16);
// assertTrue(function.checkTheoreticalStartCost(optimizer.getRMS()));
try {
VectorialPointValuePair optimum =
optimizer.optimize(function,
function.getTarget(), function.getWeight(),
function.getStartPoint());
assertFalse(exceptionExpected);
function.checkTheoreticalMinCost(optimizer.getRMS());
function.checkTheoreticalMinParams(optimum);
} catch (OptimizationException lsse) {
assertTrue(exceptionExpected);
} catch (FunctionEvaluationException fe) {
assertTrue(exceptionExpected);
}
}
private static abstract class MinpackFunction
implements DifferentiableMultivariateVectorialFunction, Serializable {
private static final long serialVersionUID = -6209760235478794233L;
protected int n;
protected int m;
protected double[] startParams;
protected double theoreticalMinCost;
protected double[] theoreticalMinParams;
protected double costAccuracy;
protected double paramsAccuracy;
protected MinpackFunction(int m, double[] startParams,
double theoreticalMinCost, double[] theoreticalMinParams) {
this.m = m;
this.n = startParams.length;
this.startParams = startParams.clone();
this.theoreticalMinCost = theoreticalMinCost;
this.theoreticalMinParams = theoreticalMinParams;
this.costAccuracy = 1.0e-8;
this.paramsAccuracy = 1.0e-5;
}
protected static double[] buildArray(int n, double x) {
double[] array = new double[n];
Arrays.fill(array, x);
return array;
}
public double[] getTarget() {
return buildArray(m, 0.0);
}
public double[] getWeight() {
return buildArray(m, 1.0);
}
public double[] getStartPoint() {
return startParams.clone();
}
protected void setCostAccuracy(double costAccuracy) {
this.costAccuracy = costAccuracy;
}
protected void setParamsAccuracy(double paramsAccuracy) {
this.paramsAccuracy = paramsAccuracy;
}
public int getN() {
return startParams.length;
}
public void checkTheoreticalMinCost(double rms) {
double threshold = costAccuracy * (1.0 + theoreticalMinCost);
assertEquals(theoreticalMinCost, Math.sqrt(m) * rms, threshold);
}
public void checkTheoreticalMinParams(VectorialPointValuePair optimum) {
double[] params = optimum.getPointRef();
if (theoreticalMinParams != null) {
for (int i = 0; i < theoreticalMinParams.length; ++i) {
double mi = theoreticalMinParams[i];
double vi = params[i];
assertEquals(mi, vi, paramsAccuracy * (1.0 + Math.abs(mi)));
}
}
}
public MultivariateMatrixFunction jacobian() {
return new MultivariateMatrixFunction() {
private static final long serialVersionUID = -2435076097232923678L;
public double[][] value(double[] point) {
return jacobian(point);
}
};
}
public abstract double[][] jacobian(double[] variables);
public abstract double[] value(double[] variables);
}
private static class LinearFullRankFunction extends MinpackFunction {
private static final long serialVersionUID = -9030323226268039536L;
public LinearFullRankFunction(int m, int n, double x0,
double theoreticalStartCost,
double theoreticalMinCost) {
super(m, buildArray(n, x0), theoreticalMinCost,
buildArray(n, -1.0));
}
@Override
public double[][] jacobian(double[] variables) {
double t = 2.0 / m;
double[][] jacobian = new double[m][];
for (int i = 0; i < m; ++i) {
jacobian[i] = new double[n];
for (int j = 0; j < n; ++j) {
jacobian[i][j] = (i == j) ? (1 - t) : -t;
}
}
return jacobian;
}
@Override
public double[] value(double[] variables) {
double sum = 0;
for (int i = 0; i < n; ++i) {
sum += variables[i];
}
double t = 1 + 2 * sum / m;
double[] f = new double[m];
for (int i = 0; i < n; ++i) {
f[i] = variables[i] - t;
}
Arrays.fill(f, n, m, -t);
return f;
}
}
private static class LinearRank1Function extends MinpackFunction {
private static final long serialVersionUID = 8494863245104608300L;
public LinearRank1Function(int m, int n, double x0,
double theoreticalStartCost,
double theoreticalMinCost) {
super(m, buildArray(n, x0), theoreticalMinCost, null);
}
@Override
public double[][] jacobian(double[] variables) {
double[][] jacobian = new double[m][];
for (int i = 0; i < m; ++i) {
jacobian[i] = new double[n];
for (int j = 0; j < n; ++j) {
jacobian[i][j] = (i + 1) * (j + 1);
}
}
return jacobian;
}
@Override
public double[] value(double[] variables) {
double[] f = new double[m];
double sum = 0;
for (int i = 0; i < n; ++i) {
sum += (i + 1) * variables[i];
}
for (int i = 0; i < m; ++i) {
f[i] = (i + 1) * sum - 1;
}
return f;
}
}
private static class LinearRank1ZeroColsAndRowsFunction extends MinpackFunction {
private static final long serialVersionUID = -3316653043091995018L;
public LinearRank1ZeroColsAndRowsFunction(int m, int n, double x0) {
super(m, buildArray(n, x0),
Math.sqrt((m * (m + 3) - 6) / (2.0 * (2 * m - 3))),
null);
}
@Override
public double[][] jacobian(double[] variables) {
double[][] jacobian = new double[m][];
for (int i = 0; i < m; ++i) {
jacobian[i] = new double[n];
jacobian[i][0] = 0;
for (int j = 1; j < (n - 1); ++j) {
if (i == 0) {
jacobian[i][j] = 0;
} else if (i != (m - 1)) {
jacobian[i][j] = i * (j + 1);
} else {
jacobian[i][j] = 0;
}
}
jacobian[i][n - 1] = 0;
}
return jacobian;
}
@Override
public double[] value(double[] variables) {
double[] f = new double[m];
double sum = 0;
for (int i = 1; i < (n - 1); ++i) {
sum += (i + 1) * variables[i];
}
for (int i = 0; i < (m - 1); ++i) {
f[i] = i * sum - 1;
}
f[m - 1] = -1;
return f;
}
}
private static class RosenbrockFunction extends MinpackFunction {
private static final long serialVersionUID = 2893438180956569134L;
public RosenbrockFunction(double[] startParams, double theoreticalStartCost) {
super(2, startParams, 0.0, buildArray(2, 1.0));
}
@Override
public double[][] jacobian(double[] variables) {
double x1 = variables[0];
return new double[][] { { -20 * x1, 10 }, { -1, 0 } };
}
@Override
public double[] value(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
return new double[] { 10 * (x2 - x1 * x1), 1 - x1 };
}
}
private static class HelicalValleyFunction extends MinpackFunction {
private static final long serialVersionUID = 220613787843200102L;
public HelicalValleyFunction(double[] startParams,
double theoreticalStartCost) {
super(3, startParams, 0.0, new double[] { 1.0, 0.0, 0.0 });
}
@Override
public double[][] jacobian(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
double tmpSquare = x1 * x1 + x2 * x2;
double tmp1 = twoPi * tmpSquare;
double tmp2 = Math.sqrt(tmpSquare);
return new double[][] {
{ 100 * x2 / tmp1, -100 * x1 / tmp1, 10 },
{ 10 * x1 / tmp2, 10 * x2 / tmp2, 0 },
{ 0, 0, 1 }
};
}
@Override
public double[] value(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
double x3 = variables[2];
double tmp1;
if (x1 == 0) {
tmp1 = (x2 >= 0) ? 0.25 : -0.25;
} else {
tmp1 = Math.atan(x2 / x1) / twoPi;
if (x1 < 0) {
tmp1 += 0.5;
}
}
double tmp2 = Math.sqrt(x1 * x1 + x2 * x2);
return new double[] {
10.0 * (x3 - 10 * tmp1),
10.0 * (tmp2 - 1),
x3
};
}
private static final double twoPi = 2.0 * Math.PI;
}
private static class PowellSingularFunction extends MinpackFunction {
private static final long serialVersionUID = 7298364171208142405L;
public PowellSingularFunction(double[] startParams,
double theoreticalStartCost) {
super(4, startParams, 0.0, buildArray(4, 0.0));
}
@Override
public double[][] jacobian(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
double x3 = variables[2];
double x4 = variables[3];
return new double[][] {
{ 1, 10, 0, 0 },
{ 0, 0, sqrt5, -sqrt5 },
{ 0, 2 * (x2 - 2 * x3), -4 * (x2 - 2 * x3), 0 },
{ 2 * sqrt10 * (x1 - x4), 0, 0, -2 * sqrt10 * (x1 - x4) }
};
}
@Override
public double[] value(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
double x3 = variables[2];
double x4 = variables[3];
return new double[] {
x1 + 10 * x2,
sqrt5 * (x3 - x4),
(x2 - 2 * x3) * (x2 - 2 * x3),
sqrt10 * (x1 - x4) * (x1 - x4)
};
}
private static final double sqrt5 = Math.sqrt( 5.0);
private static final double sqrt10 = Math.sqrt(10.0);
}
private static class FreudensteinRothFunction extends MinpackFunction {
private static final long serialVersionUID = 2892404999344244214L;
public FreudensteinRothFunction(double[] startParams,
double theoreticalStartCost,
double theoreticalMinCost,
double[] theoreticalMinParams) {
super(2, startParams, theoreticalMinCost,
theoreticalMinParams);
}
@Override
public double[][] jacobian(double[] variables) {
double x2 = variables[1];
return new double[][] {
{ 1, x2 * (10 - 3 * x2) - 2 },
{ 1, x2 * ( 2 + 3 * x2) - 14, }
};
}
@Override
public double[] value(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
return new double[] {
-13.0 + x1 + ((5.0 - x2) * x2 - 2.0) * x2,
-29.0 + x1 + ((1.0 + x2) * x2 - 14.0) * x2
};
}
}
private static class BardFunction extends MinpackFunction {
private static final long serialVersionUID = 5990442612572087668L;
public BardFunction(double x0,
double theoreticalStartCost,
double theoreticalMinCost,
double[] theoreticalMinParams) {
super(15, buildArray(3, x0), theoreticalMinCost,
theoreticalMinParams);
}
@Override
public double[][] jacobian(double[] variables) {
double x2 = variables[1];
double x3 = variables[2];
double[][] jacobian = new double[m][];
for (int i = 0; i < m; ++i) {
double tmp1 = i + 1;
double tmp2 = 15 - i;
double tmp3 = (i <= 7) ? tmp1 : tmp2;
double tmp4 = x2 * tmp2 + x3 * tmp3;
tmp4 *= tmp4;
jacobian[i] = new double[] { -1, tmp1 * tmp2 / tmp4, tmp1 * tmp3 / tmp4 };
}
return jacobian;
}
@Override
public double[] value(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
double x3 = variables[2];
double[] f = new double[m];
for (int i = 0; i < m; ++i) {
double tmp1 = i + 1;
double tmp2 = 15 - i;
double tmp3 = (i <= 7) ? tmp1 : tmp2;
f[i] = y[i] - (x1 + tmp1 / (x2 * tmp2 + x3 * tmp3));
}
return f;
}
private static final double[] y = {
0.14, 0.18, 0.22, 0.25, 0.29,
0.32, 0.35, 0.39, 0.37, 0.58,
0.73, 0.96, 1.34, 2.10, 4.39
};
}
private static class KowalikOsborneFunction extends MinpackFunction {
private static final long serialVersionUID = -4867445739880495801L;
public KowalikOsborneFunction(double[] startParams,
double theoreticalStartCost,
double theoreticalMinCost,
double[] theoreticalMinParams) {
super(11, startParams, theoreticalMinCost,
theoreticalMinParams);
if (theoreticalStartCost > 20.0) {
setCostAccuracy(2.0e-4);
setParamsAccuracy(5.0e-3);
}
}
@Override
public double[][] jacobian(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
double x3 = variables[2];
double x4 = variables[3];
double[][] jacobian = new double[m][];
for (int i = 0; i < m; ++i) {
double tmp = v[i] * (v[i] + x3) + x4;
double j1 = -v[i] * (v[i] + x2) / tmp;
double j2 = -v[i] * x1 / tmp;
double j3 = j1 * j2;
double j4 = j3 / v[i];
jacobian[i] = new double[] { j1, j2, j3, j4 };
}
return jacobian;
}
@Override
public double[] value(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
double x3 = variables[2];
double x4 = variables[3];
double[] f = new double[m];
for (int i = 0; i < m; ++i) {
f[i] = y[i] - x1 * (v[i] * (v[i] + x2)) / (v[i] * (v[i] + x3) + x4);
}
return f;
}
private static final double[] v = {
4.0, 2.0, 1.0, 0.5, 0.25, 0.167, 0.125, 0.1, 0.0833, 0.0714, 0.0625
};
private static final double[] y = {
0.1957, 0.1947, 0.1735, 0.1600, 0.0844, 0.0627,
0.0456, 0.0342, 0.0323, 0.0235, 0.0246
};
}
private static class MeyerFunction extends MinpackFunction {
private static final long serialVersionUID = -838060619150131027L;
public MeyerFunction(double[] startParams,
double theoreticalStartCost,
double theoreticalMinCost,
double[] theoreticalMinParams) {
super(16, startParams, theoreticalMinCost,
theoreticalMinParams);
if (theoreticalStartCost > 1.0e6) {
setCostAccuracy(7.0e-3);
setParamsAccuracy(2.0e-2);
}
}
@Override
public double[][] jacobian(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
double x3 = variables[2];
double[][] jacobian = new double[m][];
for (int i = 0; i < m; ++i) {
double temp = 5.0 * (i + 1) + 45.0 + x3;
double tmp1 = x2 / temp;
double tmp2 = Math.exp(tmp1);
double tmp3 = x1 * tmp2 / temp;
jacobian[i] = new double[] { tmp2, tmp3, -tmp1 * tmp3 };
}
return jacobian;
}
@Override
public double[] value(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
double x3 = variables[2];
double[] f = new double[m];
for (int i = 0; i < m; ++i) {
f[i] = x1 * Math.exp(x2 / (5.0 * (i + 1) + 45.0 + x3)) - y[i];
}
return f;
}
private static final double[] y = {
34780.0, 28610.0, 23650.0, 19630.0,
16370.0, 13720.0, 11540.0, 9744.0,
8261.0, 7030.0, 6005.0, 5147.0,
4427.0, 3820.0, 3307.0, 2872.0
};
}
private static class WatsonFunction extends MinpackFunction {
private static final long serialVersionUID = -9034759294980218927L;
public WatsonFunction(int n, double x0,
double theoreticalStartCost,
double theoreticalMinCost,
double[] theoreticalMinParams) {
super(31, buildArray(n, x0), theoreticalMinCost,
theoreticalMinParams);
}
@Override
public double[][] jacobian(double[] variables) {
double[][] jacobian = new double[m][];
for (int i = 0; i < (m - 2); ++i) {
double div = (i + 1) / 29.0;
double s2 = 0.0;
double dx = 1.0;
for (int j = 0; j < n; ++j) {
s2 += dx * variables[j];
dx *= div;
}
double temp= 2 * div * s2;
dx = 1.0 / div;
jacobian[i] = new double[n];
for (int j = 0; j < n; ++j) {
jacobian[i][j] = dx * (j - temp);
dx *= div;
}
}
jacobian[m - 2] = new double[n];
jacobian[m - 2][0] = 1;
jacobian[m - 1] = new double[n];
jacobian[m - 1][0]= -2 * variables[0];
jacobian[m - 1][1]= 1;
return jacobian;
}
@Override
public double[] value(double[] variables) {
double[] f = new double[m];
for (int i = 0; i < (m - 2); ++i) {
double div = (i + 1) / 29.0;
double s1 = 0;
double dx = 1;
for (int j = 1; j < n; ++j) {
s1 += j * dx * variables[j];
dx *= div;
}
double s2 =0;
dx =1;
for (int j = 0; j < n; ++j) {
s2 += dx * variables[j];
dx *= div;
}
f[i] = s1 - s2 * s2 - 1;
}
double x1 = variables[0];
double x2 = variables[1];
f[m - 2] = x1;
f[m - 1] = x2 - x1 * x1 - 1;
return f;
}
}
private static class Box3DimensionalFunction extends MinpackFunction {
private static final long serialVersionUID = 5511403858142574493L;
public Box3DimensionalFunction(int m, double[] startParams,
double theoreticalStartCost) {
super(m, startParams, 0.0,
new double[] { 1.0, 10.0, 1.0 });
}
@Override
public double[][] jacobian(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
double[][] jacobian = new double[m][];
for (int i = 0; i < m; ++i) {
double tmp = (i + 1) / 10.0;
jacobian[i] = new double[] {
-tmp * Math.exp(-tmp * x1),
tmp * Math.exp(-tmp * x2),
Math.exp(-i - 1) - Math.exp(-tmp)
};
}
return jacobian;
}
@Override
public double[] value(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
double x3 = variables[2];
double[] f = new double[m];
for (int i = 0; i < m; ++i) {
double tmp = (i + 1) / 10.0;
f[i] = Math.exp(-tmp * x1) - Math.exp(-tmp * x2)
+ (Math.exp(-i - 1) - Math.exp(-tmp)) * x3;
}
return f;
}
}
private static class JennrichSampsonFunction extends MinpackFunction {
private static final long serialVersionUID = -2489165190443352947L;
public JennrichSampsonFunction(int m, double[] startParams,
double theoreticalStartCost,
double theoreticalMinCost,
double[] theoreticalMinParams) {
super(m, startParams, theoreticalMinCost,
theoreticalMinParams);
}
@Override
public double[][] jacobian(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
double[][] jacobian = new double[m][];
for (int i = 0; i < m; ++i) {
double t = i + 1;
jacobian[i] = new double[] { -t * Math.exp(t * x1), -t * Math.exp(t * x2) };
}
return jacobian;
}
@Override
public double[] value(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
double[] f = new double[m];
for (int i = 0; i < m; ++i) {
double temp = i + 1;
f[i] = 2 + 2 * temp - Math.exp(temp * x1) - Math.exp(temp * x2);
}
return f;
}
}
private static class BrownDennisFunction extends MinpackFunction {
private static final long serialVersionUID = 8340018645694243910L;
public BrownDennisFunction(int m, double[] startParams,
double theoreticalStartCost,
double theoreticalMinCost,
double[] theoreticalMinParams) {
super(m, startParams, theoreticalMinCost,
theoreticalMinParams);
setCostAccuracy(2.5e-8);
}
@Override
public double[][] jacobian(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
double x3 = variables[2];
double x4 = variables[3];
double[][] jacobian = new double[m][];
for (int i = 0; i < m; ++i) {
double temp = (i + 1) / 5.0;
double ti = Math.sin(temp);
double tmp1 = x1 + temp * x2 - Math.exp(temp);
double tmp2 = x3 + ti * x4 - Math.cos(temp);
jacobian[i] = new double[] {
2 * tmp1, 2 * temp * tmp1, 2 * tmp2, 2 * ti * tmp2
};
}
return jacobian;
}
@Override
public double[] value(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
double x3 = variables[2];
double x4 = variables[3];
double[] f = new double[m];
for (int i = 0; i < m; ++i) {
double temp = (i + 1) / 5.0;
double tmp1 = x1 + temp * x2 - Math.exp(temp);
double tmp2 = x3 + Math.sin(temp) * x4 - Math.cos(temp);
f[i] = tmp1 * tmp1 + tmp2 * tmp2;
}
return f;
}
}
private static class ChebyquadFunction extends MinpackFunction {
private static final long serialVersionUID = -2394877275028008594L;
private static double[] buildChebyquadArray(int n, double factor) {
double[] array = new double[n];
double inv = factor / (n + 1);
for (int i = 0; i < n; ++i) {
array[i] = (i + 1) * inv;
}
return array;
}
public ChebyquadFunction(int n, int m, double factor,
double theoreticalStartCost,
double theoreticalMinCost,
double[] theoreticalMinParams) {
super(m, buildChebyquadArray(n, factor), theoreticalMinCost,
theoreticalMinParams);
}
@Override
public double[][] jacobian(double[] variables) {
double[][] jacobian = new double[m][];
for (int i = 0; i < m; ++i) {
jacobian[i] = new double[n];
}
double dx = 1.0 / n;
for (int j = 0; j < n; ++j) {
double tmp1 = 1;
double tmp2 = 2 * variables[j] - 1;
double temp = 2 * tmp2;
double tmp3 = 0;
double tmp4 = 2;
for (int i = 0; i < m; ++i) {
jacobian[i][j] = dx * tmp4;
double ti = 4 * tmp2 + temp * tmp4 - tmp3;
tmp3 = tmp4;
tmp4 = ti;
ti = temp * tmp2 - tmp1;
tmp1 = tmp2;
tmp2 = ti;
}
}
return jacobian;
}
@Override
public double[] value(double[] variables) {
double[] f = new double[m];
for (int j = 0; j < n; ++j) {
double tmp1 = 1;
double tmp2 = 2 * variables[j] - 1;
double temp = 2 * tmp2;
for (int i = 0; i < m; ++i) {
f[i] += tmp2;
double ti = temp * tmp2 - tmp1;
tmp1 = tmp2;
tmp2 = ti;
}
}
double dx = 1.0 / n;
boolean iev = false;
for (int i = 0; i < m; ++i) {
f[i] *= dx;
if (iev) {
f[i] += 1.0 / (i * (i + 2));
}
iev = ! iev;
}
return f;
}
}
private static class BrownAlmostLinearFunction extends MinpackFunction {
private static final long serialVersionUID = 8239594490466964725L;
public BrownAlmostLinearFunction(int m, double factor,
double theoreticalStartCost,
double theoreticalMinCost,
double[] theoreticalMinParams) {
super(m, buildArray(m, factor), theoreticalMinCost,
theoreticalMinParams);
}
@Override
public double[][] jacobian(double[] variables) {
double[][] jacobian = new double[m][];
for (int i = 0; i < m; ++i) {
jacobian[i] = new double[n];
}
double prod = 1;
for (int j = 0; j < n; ++j) {
prod *= variables[j];
for (int i = 0; i < n; ++i) {
jacobian[i][j] = 1;
}
jacobian[j][j] = 2;
}
for (int j = 0; j < n; ++j) {
double temp = variables[j];
if (temp == 0) {
temp = 1;
prod = 1;
for (int k = 0; k < n; ++k) {
if (k != j) {
prod *= variables[k];
}
}
}
jacobian[n - 1][j] = prod / temp;
}
return jacobian;
}
@Override
public double[] value(double[] variables) {
double[] f = new double[m];
double sum = -(n + 1);
double prod = 1;
for (int j = 0; j < n; ++j) {
sum += variables[j];
prod *= variables[j];
}
for (int i = 0; i < n; ++i) {
f[i] = variables[i] + sum;
}
f[n - 1] = prod - 1;
return f;
}
}
private static class Osborne1Function extends MinpackFunction {
private static final long serialVersionUID = 4006743521149849494L;
public Osborne1Function(double[] startParams,
double theoreticalStartCost,
double theoreticalMinCost,
double[] theoreticalMinParams) {
super(33, startParams, theoreticalMinCost,
theoreticalMinParams);
}
@Override
public double[][] jacobian(double[] variables) {
double x2 = variables[1];
double x3 = variables[2];
double x4 = variables[3];
double x5 = variables[4];
double[][] jacobian = new double[m][];
for (int i = 0; i < m; ++i) {
double temp = 10.0 * i;
double tmp1 = Math.exp(-temp * x4);
double tmp2 = Math.exp(-temp * x5);
jacobian[i] = new double[] {
-1, -tmp1, -tmp2, temp * x2 * tmp1, temp * x3 * tmp2
};
}
return jacobian;
}
@Override
public double[] value(double[] variables) {
double x1 = variables[0];
double x2 = variables[1];
double x3 = variables[2];
double x4 = variables[3];
double x5 = variables[4];
double[] f = new double[m];
for (int i = 0; i < m; ++i) {
double temp = 10.0 * i;
double tmp1 = Math.exp(-temp * x4);
double tmp2 = Math.exp(-temp * x5);
f[i] = y[i] - (x1 + x2 * tmp1 + x3 * tmp2);
}
return f;
}
private static final double[] y = {
0.844, 0.908, 0.932, 0.936, 0.925, 0.908, 0.881, 0.850, 0.818, 0.784, 0.751,
0.718, 0.685, 0.658, 0.628, 0.603, 0.580, 0.558, 0.538, 0.522, 0.506, 0.490,
0.478, 0.467, 0.457, 0.448, 0.438, 0.431, 0.424, 0.420, 0.414, 0.411, 0.406
};
}
private static class Osborne2Function extends MinpackFunction {
private static final long serialVersionUID = -8418268780389858746L;
public Osborne2Function(double[] startParams,
double theoreticalStartCost,
double theoreticalMinCost,
double[] theoreticalMinParams) {
super(65, startParams, theoreticalMinCost,
theoreticalMinParams);
}
@Override
public double[][] jacobian(double[] variables) {
double x01 = variables[0];
double x02 = variables[1];
double x03 = variables[2];
double x04 = variables[3];
double x05 = variables[4];
double x06 = variables[5];
double x07 = variables[6];
double x08 = variables[7];
double x09 = variables[8];
double x10 = variables[9];
double x11 = variables[10];
double[][] jacobian = new double[m][];
for (int i = 0; i < m; ++i) {
double temp = i / 10.0;
double tmp1 = Math.exp(-x05 * temp);
double tmp2 = Math.exp(-x06 * (temp - x09) * (temp - x09));
double tmp3 = Math.exp(-x07 * (temp - x10) * (temp - x10));
double tmp4 = Math.exp(-x08 * (temp - x11) * (temp - x11));
jacobian[i] = new double[] {
-tmp1,
-tmp2,
-tmp3,
-tmp4,
temp * x01 * tmp1,
x02 * (temp - x09) * (temp - x09) * tmp2,
x03 * (temp - x10) * (temp - x10) * tmp3,
x04 * (temp - x11) * (temp - x11) * tmp4,
-2 * x02 * x06 * (temp - x09) * tmp2,
-2 * x03 * x07 * (temp - x10) * tmp3,
-2 * x04 * x08 * (temp - x11) * tmp4
};
}
return jacobian;
}
@Override
public double[] value(double[] variables) {
double x01 = variables[0];
double x02 = variables[1];
double x03 = variables[2];
double x04 = variables[3];
double x05 = variables[4];
double x06 = variables[5];
double x07 = variables[6];
double x08 = variables[7];
double x09 = variables[8];
double x10 = variables[9];
double x11 = variables[10];
double[] f = new double[m];
for (int i = 0; i < m; ++i) {
double temp = i / 10.0;
double tmp1 = Math.exp(-x05 * temp);
double tmp2 = Math.exp(-x06 * (temp - x09) * (temp - x09));
double tmp3 = Math.exp(-x07 * (temp - x10) * (temp - x10));
double tmp4 = Math.exp(-x08 * (temp - x11) * (temp - x11));
f[i] = y[i] - (x01 * tmp1 + x02 * tmp2 + x03 * tmp3 + x04 * tmp4);
}
return f;
}
private static final double[] y = {
1.366, 1.191, 1.112, 1.013, 0.991,
0.885, 0.831, 0.847, 0.786, 0.725,
0.746, 0.679, 0.608, 0.655, 0.616,
0.606, 0.602, 0.626, 0.651, 0.724,
0.649, 0.649, 0.694, 0.644, 0.624,
0.661, 0.612, 0.558, 0.533, 0.495,
0.500, 0.423, 0.395, 0.375, 0.372,
0.391, 0.396, 0.405, 0.428, 0.429,
0.523, 0.562, 0.607, 0.653, 0.672,
0.708, 0.633, 0.668, 0.645, 0.632,
0.591, 0.559, 0.597, 0.625, 0.739,
0.710, 0.729, 0.720, 0.636, 0.581,
0.428, 0.292, 0.162, 0.098, 0.054
};
}
} |
||
@Test
public void testToMapWithNoHeader() throws Exception {
final CSVParser parser = CSVParser.parse("a,b", CSVFormat.newFormat(','));
final CSVRecord shortRec = parser.iterator().next();
Map<String, String> map = shortRec.toMap();
assertNotNull("Map is not null.", map);
assertTrue("Map is empty.", map.isEmpty());
} | org.apache.commons.csv.CSVRecordTest::testToMapWithNoHeader | src/test/java/org/apache/commons/csv/CSVRecordTest.java | 177 | src/test/java/org/apache/commons/csv/CSVRecordTest.java | testToMapWithNoHeader | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.csv;
import static org.junit.Assert.assertEquals;
import static org.junit.Assert.assertFalse;
import static org.junit.Assert.assertNotNull;
import static org.junit.Assert.assertNull;
import static org.junit.Assert.assertTrue;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.TreeMap;
import java.util.concurrent.ConcurrentHashMap;
import org.junit.Assert;
import org.junit.Before;
import org.junit.Test;
public class CSVRecordTest {
private enum EnumFixture { UNKNOWN_COLUMN }
private String[] values;
private CSVRecord record, recordWithHeader;
private Map<String, Integer> header;
@Before
public void setUp() throws Exception {
values = new String[] { "A", "B", "C" };
record = new CSVRecord(values, null, null, 0);
header = new HashMap<String, Integer>();
header.put("first", Integer.valueOf(0));
header.put("second", Integer.valueOf(1));
header.put("third", Integer.valueOf(2));
recordWithHeader = new CSVRecord(values, header, null, 0);
}
@Test
public void testGetInt() {
assertEquals(values[0], record.get(0));
assertEquals(values[1], record.get(1));
assertEquals(values[2], record.get(2));
}
@Test
public void testGetString() {
assertEquals(values[0], recordWithHeader.get("first"));
assertEquals(values[1], recordWithHeader.get("second"));
assertEquals(values[2], recordWithHeader.get("third"));
}
@Test(expected = IllegalArgumentException.class)
public void testGetStringInconsistentRecord() {
header.put("fourth", Integer.valueOf(4));
recordWithHeader.get("fourth");
}
@Test(expected = IllegalStateException.class)
public void testGetStringNoHeader() {
record.get("first");
}
@Test(expected = IllegalArgumentException.class)
public void testGetUnmappedEnum() {
assertNull(recordWithHeader.get(EnumFixture.UNKNOWN_COLUMN));
}
@Test(expected = IllegalArgumentException.class)
public void testGetUnmappedName() {
assertNull(recordWithHeader.get("fourth"));
}
@Test(expected = ArrayIndexOutOfBoundsException.class)
public void testGetUnmappedNegativeInt() {
assertNull(recordWithHeader.get(Integer.MIN_VALUE));
}
@Test(expected = ArrayIndexOutOfBoundsException.class)
public void testGetUnmappedPositiveInt() {
assertNull(recordWithHeader.get(Integer.MAX_VALUE));
}
@Test
public void testIsConsistent() {
assertTrue(record.isConsistent());
assertTrue(recordWithHeader.isConsistent());
header.put("fourth", Integer.valueOf(4));
assertFalse(recordWithHeader.isConsistent());
}
@Test
public void testIsMapped() {
assertFalse(record.isMapped("first"));
assertTrue(recordWithHeader.isMapped("first"));
assertFalse(recordWithHeader.isMapped("fourth"));
}
@Test
public void testIsSet() {
assertFalse(record.isSet("first"));
assertTrue(recordWithHeader.isSet("first"));
assertFalse(recordWithHeader.isSet("fourth"));
}
@Test
public void testIterator() {
int i = 0;
for (final String value : record) {
assertEquals(values[i], value);
i++;
}
}
@Test
public void testPutInMap() {
final Map<String, String> map = new ConcurrentHashMap<String, String>();
this.recordWithHeader.putIn(map);
this.validateMap(map, false);
// Test that we can compile with assigment to the same map as the param.
final TreeMap<String, String> map2 = recordWithHeader.putIn(new TreeMap<String, String>());
this.validateMap(map2, false);
}
@Test
public void testRemoveAndAddColumns() throws IOException {
// do:
final CSVPrinter printer = new CSVPrinter(new StringBuilder(), CSVFormat.DEFAULT);
final Map<String, String> map = recordWithHeader.toMap();
map.remove("OldColumn");
map.put("ZColumn", "NewValue");
// check:
final ArrayList<String> list = new ArrayList<String>(map.values());
Collections.sort(list);
printer.printRecord(list);
Assert.assertEquals("A,B,C,NewValue" + CSVFormat.DEFAULT.getRecordSeparator(), printer.getOut().toString());
printer.close();
}
@Test
public void testToMap() {
final Map<String, String> map = this.recordWithHeader.toMap();
this.validateMap(map, true);
}
@Test
public void testToMapWithShortRecord() throws Exception {
final CSVParser parser = CSVParser.parse("a,b", CSVFormat.DEFAULT.withHeader("A", "B", "C"));
final CSVRecord shortRec = parser.iterator().next();
shortRec.toMap();
}
@Test
public void testToMapWithNoHeader() throws Exception {
final CSVParser parser = CSVParser.parse("a,b", CSVFormat.newFormat(','));
final CSVRecord shortRec = parser.iterator().next();
Map<String, String> map = shortRec.toMap();
assertNotNull("Map is not null.", map);
assertTrue("Map is empty.", map.isEmpty());
}
private void validateMap(final Map<String, String> map, final boolean allowsNulls) {
assertTrue(map.containsKey("first"));
assertTrue(map.containsKey("second"));
assertTrue(map.containsKey("third"));
assertFalse(map.containsKey("fourth"));
if (allowsNulls) {
assertFalse(map.containsKey(null));
}
assertEquals("A", map.get("first"));
assertEquals("B", map.get("second"));
assertEquals("C", map.get("third"));
assertEquals(null, map.get("fourth"));
}
} | // You are a professional Java test case writer, please create a test case named `testToMapWithNoHeader` for the issue `Csv-CSV-118`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Csv-CSV-118
//
// ## Issue-Title:
// CSVRecord.toMap() throws NPE on formats with no headers.
//
// ## Issue-Description:
//
// The method toMap() on CSVRecord throws a NullPointerExcpetion when called on records derived using a format with no headers.
//
//
// The method documentation states a null map should be returned instead.
//
//
//
//
//
@Test
public void testToMapWithNoHeader() throws Exception {
| 177 | 9 | 170 | src/test/java/org/apache/commons/csv/CSVRecordTest.java | src/test/java | ```markdown
## Issue-ID: Csv-CSV-118
## Issue-Title:
CSVRecord.toMap() throws NPE on formats with no headers.
## Issue-Description:
The method toMap() on CSVRecord throws a NullPointerExcpetion when called on records derived using a format with no headers.
The method documentation states a null map should be returned instead.
```
You are a professional Java test case writer, please create a test case named `testToMapWithNoHeader` for the issue `Csv-CSV-118`, utilizing the provided issue report information and the following function signature.
```java
@Test
public void testToMapWithNoHeader() throws Exception {
```
| 170 | [
"org.apache.commons.csv.CSVRecord"
] | 7fbb9d544e8425193e189642ad0230a65c0068033fa623b6193681ad02bf05c2 | @Test
public void testToMapWithNoHeader() throws Exception | // You are a professional Java test case writer, please create a test case named `testToMapWithNoHeader` for the issue `Csv-CSV-118`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Csv-CSV-118
//
// ## Issue-Title:
// CSVRecord.toMap() throws NPE on formats with no headers.
//
// ## Issue-Description:
//
// The method toMap() on CSVRecord throws a NullPointerExcpetion when called on records derived using a format with no headers.
//
//
// The method documentation states a null map should be returned instead.
//
//
//
//
//
| Csv | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.csv;
import static org.junit.Assert.assertEquals;
import static org.junit.Assert.assertFalse;
import static org.junit.Assert.assertNotNull;
import static org.junit.Assert.assertNull;
import static org.junit.Assert.assertTrue;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.TreeMap;
import java.util.concurrent.ConcurrentHashMap;
import org.junit.Assert;
import org.junit.Before;
import org.junit.Test;
public class CSVRecordTest {
private enum EnumFixture { UNKNOWN_COLUMN }
private String[] values;
private CSVRecord record, recordWithHeader;
private Map<String, Integer> header;
@Before
public void setUp() throws Exception {
values = new String[] { "A", "B", "C" };
record = new CSVRecord(values, null, null, 0);
header = new HashMap<String, Integer>();
header.put("first", Integer.valueOf(0));
header.put("second", Integer.valueOf(1));
header.put("third", Integer.valueOf(2));
recordWithHeader = new CSVRecord(values, header, null, 0);
}
@Test
public void testGetInt() {
assertEquals(values[0], record.get(0));
assertEquals(values[1], record.get(1));
assertEquals(values[2], record.get(2));
}
@Test
public void testGetString() {
assertEquals(values[0], recordWithHeader.get("first"));
assertEquals(values[1], recordWithHeader.get("second"));
assertEquals(values[2], recordWithHeader.get("third"));
}
@Test(expected = IllegalArgumentException.class)
public void testGetStringInconsistentRecord() {
header.put("fourth", Integer.valueOf(4));
recordWithHeader.get("fourth");
}
@Test(expected = IllegalStateException.class)
public void testGetStringNoHeader() {
record.get("first");
}
@Test(expected = IllegalArgumentException.class)
public void testGetUnmappedEnum() {
assertNull(recordWithHeader.get(EnumFixture.UNKNOWN_COLUMN));
}
@Test(expected = IllegalArgumentException.class)
public void testGetUnmappedName() {
assertNull(recordWithHeader.get("fourth"));
}
@Test(expected = ArrayIndexOutOfBoundsException.class)
public void testGetUnmappedNegativeInt() {
assertNull(recordWithHeader.get(Integer.MIN_VALUE));
}
@Test(expected = ArrayIndexOutOfBoundsException.class)
public void testGetUnmappedPositiveInt() {
assertNull(recordWithHeader.get(Integer.MAX_VALUE));
}
@Test
public void testIsConsistent() {
assertTrue(record.isConsistent());
assertTrue(recordWithHeader.isConsistent());
header.put("fourth", Integer.valueOf(4));
assertFalse(recordWithHeader.isConsistent());
}
@Test
public void testIsMapped() {
assertFalse(record.isMapped("first"));
assertTrue(recordWithHeader.isMapped("first"));
assertFalse(recordWithHeader.isMapped("fourth"));
}
@Test
public void testIsSet() {
assertFalse(record.isSet("first"));
assertTrue(recordWithHeader.isSet("first"));
assertFalse(recordWithHeader.isSet("fourth"));
}
@Test
public void testIterator() {
int i = 0;
for (final String value : record) {
assertEquals(values[i], value);
i++;
}
}
@Test
public void testPutInMap() {
final Map<String, String> map = new ConcurrentHashMap<String, String>();
this.recordWithHeader.putIn(map);
this.validateMap(map, false);
// Test that we can compile with assigment to the same map as the param.
final TreeMap<String, String> map2 = recordWithHeader.putIn(new TreeMap<String, String>());
this.validateMap(map2, false);
}
@Test
public void testRemoveAndAddColumns() throws IOException {
// do:
final CSVPrinter printer = new CSVPrinter(new StringBuilder(), CSVFormat.DEFAULT);
final Map<String, String> map = recordWithHeader.toMap();
map.remove("OldColumn");
map.put("ZColumn", "NewValue");
// check:
final ArrayList<String> list = new ArrayList<String>(map.values());
Collections.sort(list);
printer.printRecord(list);
Assert.assertEquals("A,B,C,NewValue" + CSVFormat.DEFAULT.getRecordSeparator(), printer.getOut().toString());
printer.close();
}
@Test
public void testToMap() {
final Map<String, String> map = this.recordWithHeader.toMap();
this.validateMap(map, true);
}
@Test
public void testToMapWithShortRecord() throws Exception {
final CSVParser parser = CSVParser.parse("a,b", CSVFormat.DEFAULT.withHeader("A", "B", "C"));
final CSVRecord shortRec = parser.iterator().next();
shortRec.toMap();
}
@Test
public void testToMapWithNoHeader() throws Exception {
final CSVParser parser = CSVParser.parse("a,b", CSVFormat.newFormat(','));
final CSVRecord shortRec = parser.iterator().next();
Map<String, String> map = shortRec.toMap();
assertNotNull("Map is not null.", map);
assertTrue("Map is empty.", map.isEmpty());
}
private void validateMap(final Map<String, String> map, final boolean allowsNulls) {
assertTrue(map.containsKey("first"));
assertTrue(map.containsKey("second"));
assertTrue(map.containsKey("third"));
assertFalse(map.containsKey("fourth"));
if (allowsNulls) {
assertFalse(map.containsKey(null));
}
assertEquals("A", map.get("first"));
assertEquals("B", map.get("second"));
assertEquals("C", map.get("third"));
assertEquals(null, map.get("fourth"));
}
} |
||
public void testRoundTripNames(){
checkName("");
checkName("The quick brown fox\n");
checkName("\177");
// checkName("\0"); // does not work, because NUL is ignored
// COMPRESS-114
checkName("0302-0601-3±±±F06±W220±ZB±LALALA±±±±±±±±±±CAN±±DC±±±04±060302±MOE.model");
} | org.apache.commons.compress.archivers.tar.TarUtilsTest::testRoundTripNames | src/test/java/org/apache/commons/compress/archivers/tar/TarUtilsTest.java | 126 | src/test/java/org/apache/commons/compress/archivers/tar/TarUtilsTest.java | testRoundTripNames | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package org.apache.commons.compress.archivers.tar;
import junit.framework.TestCase;
public class TarUtilsTest extends TestCase {
public void testName(){
byte [] buff = new byte[20];
String sb1 = "abcdefghijklmnopqrstuvwxyz";
int off = TarUtils.formatNameBytes(sb1, buff, 1, buff.length-1);
assertEquals(off, 20);
String sb2 = TarUtils.parseName(buff, 1, 10);
assertEquals(sb2,sb1.substring(0,10));
sb2 = TarUtils.parseName(buff, 1, 19);
assertEquals(sb2,sb1.substring(0,19));
buff = new byte[30];
off = TarUtils.formatNameBytes(sb1, buff, 1, buff.length-1);
assertEquals(off, 30);
sb2 = TarUtils.parseName(buff, 1, buff.length-1);
assertEquals(sb1, sb2);
}
private void fillBuff(byte []buffer, String input) throws Exception{
for(int i=0; i<buffer.length;i++){
buffer[i]=0;
}
System.arraycopy(input.getBytes("UTF-8"),0,buffer,0,Math.min(buffer.length,input.length()));
}
public void testParseOctal() throws Exception{
byte [] buffer = new byte[20];
fillBuff(buffer,"777777777777 ");
long value;
value = TarUtils.parseOctal(buffer,0, 11);
assertEquals(077777777777L, value);
value = TarUtils.parseOctal(buffer,0, 12);
assertEquals(0777777777777L, value);
buffer[11]=' ';
value = TarUtils.parseOctal(buffer,0, 11);
assertEquals(077777777777L, value);
buffer[11]=0;
value = TarUtils.parseOctal(buffer,0, 11);
assertEquals(077777777777L, value);
fillBuff(buffer, "abcdef"); // Invalid input
try {
value = TarUtils.parseOctal(buffer,0, 11);
fail("Expected IllegalArgumentException");
} catch (IllegalArgumentException expected) {
}
}
private void checkRoundTripOctal(final long value) {
byte [] buffer = new byte[12];
long parseValue;
TarUtils.formatLongOctalBytes(value, buffer, 0, buffer.length);
parseValue = TarUtils.parseOctal(buffer,0, buffer.length);
assertEquals(value,parseValue);
}
public void testRoundTripOctal() {
checkRoundTripOctal(0);
checkRoundTripOctal(1);
// checkRoundTripOctal(-1); // TODO What should this do?
checkRoundTripOctal(077777777777L);
// checkRoundTripOctal(0100000000000L); // TODO What should this do?
}
// Check correct trailing bytes are generated
public void testTrailers() {
byte [] buffer = new byte[12];
TarUtils.formatLongOctalBytes(123, buffer, 0, buffer.length);
assertEquals(' ', buffer[buffer.length-1]);
assertEquals('3', buffer[buffer.length-2]); // end of number
TarUtils.formatOctalBytes(123, buffer, 0, buffer.length);
assertEquals(0 , buffer[buffer.length-1]);
assertEquals(' ', buffer[buffer.length-2]);
assertEquals('3', buffer[buffer.length-3]); // end of number
TarUtils.formatCheckSumOctalBytes(123, buffer, 0, buffer.length);
assertEquals(' ', buffer[buffer.length-1]);
assertEquals(0 , buffer[buffer.length-2]);
assertEquals('3', buffer[buffer.length-3]); // end of number
}
public void testNegative() {
byte [] buffer = new byte[22];
TarUtils.formatUnsignedOctalString(-1, buffer, 0, buffer.length);
assertEquals("1777777777777777777777", new String(buffer));
}
public void testOverflow() {
byte [] buffer = new byte[8-1]; // a lot of the numbers have 8-byte buffers (nul term)
TarUtils.formatUnsignedOctalString(07777777L, buffer, 0, buffer.length);
assertEquals("7777777", new String(buffer));
try {
TarUtils.formatUnsignedOctalString(017777777L, buffer, 0, buffer.length);
fail("Should have cause IllegalArgumentException");
} catch (IllegalArgumentException expected) {
}
}
public void testRoundTripNames(){
checkName("");
checkName("The quick brown fox\n");
checkName("\177");
// checkName("\0"); // does not work, because NUL is ignored
// COMPRESS-114
checkName("0302-0601-3±±±F06±W220±ZB±LALALA±±±±±±±±±±CAN±±DC±±±04±060302±MOE.model");
}
private void checkName(String string) {
byte buff[] = new byte[100];
int len = TarUtils.formatNameBytes(string, buff, 0, buff.length);
assertEquals(string, TarUtils.parseName(buff, 0, len));
}
} | // You are a professional Java test case writer, please create a test case named `testRoundTripNames` for the issue `Compress-COMPRESS-114`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Compress-COMPRESS-114
//
// ## Issue-Title:
// TarUtils.parseName does not properly handle characters outside the range 0-127
//
// ## Issue-Description:
//
// if a tarfile contains files with special characters, the names of the tar entries are wrong.
//
//
// example:
//
// correct name: 0302-0601-3±±±F06±W220±ZB±LALALA±±±±±±±±±±CAN±±DC±±±04±060302±MOE.model
//
// name resolved by TarUtils.parseName: 0302-0101-3ᄆᄆᄆF06ᄆW220ᄆZBᄆHECKMODULᄆᄆᄆᄆᄆᄆᄆᄆᄆᄆECEᄆᄆDCᄆᄆᄆ07ᄆ060302ᄆDOERN.model
//
//
// please use:
//
// result.append(new String(new byte[]
//
//
// { buffer[i] }
// ));
//
//
// instead of:
//
// result.append((char) buffer[i]);
//
//
// to solve this encoding problem.
//
//
//
//
//
public void testRoundTripNames() {
| 126 | 7 | 119 | src/test/java/org/apache/commons/compress/archivers/tar/TarUtilsTest.java | src/test/java | ```markdown
## Issue-ID: Compress-COMPRESS-114
## Issue-Title:
TarUtils.parseName does not properly handle characters outside the range 0-127
## Issue-Description:
if a tarfile contains files with special characters, the names of the tar entries are wrong.
example:
correct name: 0302-0601-3±±±F06±W220±ZB±LALALA±±±±±±±±±±CAN±±DC±±±04±060302±MOE.model
name resolved by TarUtils.parseName: 0302-0101-3ᄆᄆᄆF06ᄆW220ᄆZBᄆHECKMODULᄆᄆᄆᄆᄆᄆᄆᄆᄆᄆECEᄆᄆDCᄆᄆᄆ07ᄆ060302ᄆDOERN.model
please use:
result.append(new String(new byte[]
{ buffer[i] }
));
instead of:
result.append((char) buffer[i]);
to solve this encoding problem.
```
You are a professional Java test case writer, please create a test case named `testRoundTripNames` for the issue `Compress-COMPRESS-114`, utilizing the provided issue report information and the following function signature.
```java
public void testRoundTripNames() {
```
| 119 | [
"org.apache.commons.compress.archivers.tar.TarUtils"
] | 7fbec07ca3d104171ffbcdadaaa9c40bab55c8e36767de5c86a29d767f70693e | public void testRoundTripNames() | // You are a professional Java test case writer, please create a test case named `testRoundTripNames` for the issue `Compress-COMPRESS-114`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Compress-COMPRESS-114
//
// ## Issue-Title:
// TarUtils.parseName does not properly handle characters outside the range 0-127
//
// ## Issue-Description:
//
// if a tarfile contains files with special characters, the names of the tar entries are wrong.
//
//
// example:
//
// correct name: 0302-0601-3±±±F06±W220±ZB±LALALA±±±±±±±±±±CAN±±DC±±±04±060302±MOE.model
//
// name resolved by TarUtils.parseName: 0302-0101-3ᄆᄆᄆF06ᄆW220ᄆZBᄆHECKMODULᄆᄆᄆᄆᄆᄆᄆᄆᄆᄆECEᄆᄆDCᄆᄆᄆ07ᄆ060302ᄆDOERN.model
//
//
// please use:
//
// result.append(new String(new byte[]
//
//
// { buffer[i] }
// ));
//
//
// instead of:
//
// result.append((char) buffer[i]);
//
//
// to solve this encoding problem.
//
//
//
//
//
| Compress | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package org.apache.commons.compress.archivers.tar;
import junit.framework.TestCase;
public class TarUtilsTest extends TestCase {
public void testName(){
byte [] buff = new byte[20];
String sb1 = "abcdefghijklmnopqrstuvwxyz";
int off = TarUtils.formatNameBytes(sb1, buff, 1, buff.length-1);
assertEquals(off, 20);
String sb2 = TarUtils.parseName(buff, 1, 10);
assertEquals(sb2,sb1.substring(0,10));
sb2 = TarUtils.parseName(buff, 1, 19);
assertEquals(sb2,sb1.substring(0,19));
buff = new byte[30];
off = TarUtils.formatNameBytes(sb1, buff, 1, buff.length-1);
assertEquals(off, 30);
sb2 = TarUtils.parseName(buff, 1, buff.length-1);
assertEquals(sb1, sb2);
}
private void fillBuff(byte []buffer, String input) throws Exception{
for(int i=0; i<buffer.length;i++){
buffer[i]=0;
}
System.arraycopy(input.getBytes("UTF-8"),0,buffer,0,Math.min(buffer.length,input.length()));
}
public void testParseOctal() throws Exception{
byte [] buffer = new byte[20];
fillBuff(buffer,"777777777777 ");
long value;
value = TarUtils.parseOctal(buffer,0, 11);
assertEquals(077777777777L, value);
value = TarUtils.parseOctal(buffer,0, 12);
assertEquals(0777777777777L, value);
buffer[11]=' ';
value = TarUtils.parseOctal(buffer,0, 11);
assertEquals(077777777777L, value);
buffer[11]=0;
value = TarUtils.parseOctal(buffer,0, 11);
assertEquals(077777777777L, value);
fillBuff(buffer, "abcdef"); // Invalid input
try {
value = TarUtils.parseOctal(buffer,0, 11);
fail("Expected IllegalArgumentException");
} catch (IllegalArgumentException expected) {
}
}
private void checkRoundTripOctal(final long value) {
byte [] buffer = new byte[12];
long parseValue;
TarUtils.formatLongOctalBytes(value, buffer, 0, buffer.length);
parseValue = TarUtils.parseOctal(buffer,0, buffer.length);
assertEquals(value,parseValue);
}
public void testRoundTripOctal() {
checkRoundTripOctal(0);
checkRoundTripOctal(1);
// checkRoundTripOctal(-1); // TODO What should this do?
checkRoundTripOctal(077777777777L);
// checkRoundTripOctal(0100000000000L); // TODO What should this do?
}
// Check correct trailing bytes are generated
public void testTrailers() {
byte [] buffer = new byte[12];
TarUtils.formatLongOctalBytes(123, buffer, 0, buffer.length);
assertEquals(' ', buffer[buffer.length-1]);
assertEquals('3', buffer[buffer.length-2]); // end of number
TarUtils.formatOctalBytes(123, buffer, 0, buffer.length);
assertEquals(0 , buffer[buffer.length-1]);
assertEquals(' ', buffer[buffer.length-2]);
assertEquals('3', buffer[buffer.length-3]); // end of number
TarUtils.formatCheckSumOctalBytes(123, buffer, 0, buffer.length);
assertEquals(' ', buffer[buffer.length-1]);
assertEquals(0 , buffer[buffer.length-2]);
assertEquals('3', buffer[buffer.length-3]); // end of number
}
public void testNegative() {
byte [] buffer = new byte[22];
TarUtils.formatUnsignedOctalString(-1, buffer, 0, buffer.length);
assertEquals("1777777777777777777777", new String(buffer));
}
public void testOverflow() {
byte [] buffer = new byte[8-1]; // a lot of the numbers have 8-byte buffers (nul term)
TarUtils.formatUnsignedOctalString(07777777L, buffer, 0, buffer.length);
assertEquals("7777777", new String(buffer));
try {
TarUtils.formatUnsignedOctalString(017777777L, buffer, 0, buffer.length);
fail("Should have cause IllegalArgumentException");
} catch (IllegalArgumentException expected) {
}
}
public void testRoundTripNames(){
checkName("");
checkName("The quick brown fox\n");
checkName("\177");
// checkName("\0"); // does not work, because NUL is ignored
// COMPRESS-114
checkName("0302-0601-3±±±F06±W220±ZB±LALALA±±±±±±±±±±CAN±±DC±±±04±060302±MOE.model");
}
private void checkName(String string) {
byte buff[] = new byte[100];
int len = TarUtils.formatNameBytes(string, buff, 0, buff.length);
assertEquals(string, TarUtils.parseName(buff, 0, len));
}
} |
||
@Test
public void testKeepInitIfBest() {
final double minSin = 3 * Math.PI / 2;
final double offset = 1e-8;
final double delta = 1e-7;
final UnivariateFunction f1 = new Sin();
final UnivariateFunction f2 = new StepFunction(new double[] { minSin, minSin + offset, minSin + 2 * offset},
new double[] { 0, -1, 0 });
final UnivariateFunction f = FunctionUtils.add(f1, f2);
// A slightly less stringent tolerance would make the test pass
// even with the previous implementation.
final double relTol = 1e-8;
final UnivariateOptimizer optimizer = new BrentOptimizer(relTol, 1e-100);
final double init = minSin + 1.5 * offset;
final UnivariatePointValuePair result
= optimizer.optimize(200, f, GoalType.MINIMIZE,
minSin - 6.789 * delta,
minSin + 9.876 * delta,
init);
final int numEval = optimizer.getEvaluations();
final double sol = result.getPoint();
final double expected = init;
// System.out.println("numEval=" + numEval);
// System.out.println("min=" + init + " f=" + f.value(init));
// System.out.println("sol=" + sol + " f=" + f.value(sol));
// System.out.println("exp=" + expected + " f=" + f.value(expected));
Assert.assertTrue("Best point not reported", f.value(sol) <= f.value(expected));
} | org.apache.commons.math3.optimization.univariate.BrentOptimizerTest::testKeepInitIfBest | src/test/java/org/apache/commons/math3/optimization/univariate/BrentOptimizerTest.java | 221 | src/test/java/org/apache/commons/math3/optimization/univariate/BrentOptimizerTest.java | testKeepInitIfBest | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.math3.optimization.univariate;
import org.apache.commons.math3.analysis.QuinticFunction;
import org.apache.commons.math3.analysis.UnivariateFunction;
import org.apache.commons.math3.analysis.function.Sin;
import org.apache.commons.math3.analysis.function.StepFunction;
import org.apache.commons.math3.analysis.FunctionUtils;
import org.apache.commons.math3.exception.NumberIsTooLargeException;
import org.apache.commons.math3.exception.NumberIsTooSmallException;
import org.apache.commons.math3.exception.TooManyEvaluationsException;
import org.apache.commons.math3.optimization.ConvergenceChecker;
import org.apache.commons.math3.optimization.GoalType;
import org.apache.commons.math3.stat.descriptive.DescriptiveStatistics;
import org.apache.commons.math3.util.FastMath;
import org.junit.Assert;
import org.junit.Test;
/**
* @version $Id$
*/
public final class BrentOptimizerTest {
@Test
public void testSinMin() {
UnivariateFunction f = new Sin();
UnivariateOptimizer optimizer = new BrentOptimizer(1e-10, 1e-14);
Assert.assertEquals(3 * Math.PI / 2, optimizer.optimize(200, f, GoalType.MINIMIZE, 4, 5).getPoint(), 1e-8);
Assert.assertTrue(optimizer.getEvaluations() <= 50);
Assert.assertEquals(200, optimizer.getMaxEvaluations());
Assert.assertEquals(3 * Math.PI / 2, optimizer.optimize(200, f, GoalType.MINIMIZE, 1, 5).getPoint(), 1e-8);
Assert.assertTrue(optimizer.getEvaluations() <= 100);
Assert.assertTrue(optimizer.getEvaluations() >= 15);
try {
optimizer.optimize(10, f, GoalType.MINIMIZE, 4, 5);
Assert.fail("an exception should have been thrown");
} catch (TooManyEvaluationsException fee) {
// expected
}
}
@Test
public void testSinMinWithValueChecker() {
final UnivariateFunction f = new Sin();
final ConvergenceChecker<UnivariatePointValuePair> checker = new SimpleUnivariateValueChecker(1e-5, 1e-14);
// The default stopping criterion of Brent's algorithm should not
// pass, but the search will stop at the given relative tolerance
// for the function value.
final UnivariateOptimizer optimizer = new BrentOptimizer(1e-10, 1e-14, checker);
final UnivariatePointValuePair result = optimizer.optimize(200, f, GoalType.MINIMIZE, 4, 5);
Assert.assertEquals(3 * Math.PI / 2, result.getPoint(), 1e-3);
}
@Test
public void testBoundaries() {
final double lower = -1.0;
final double upper = +1.0;
UnivariateFunction f = new UnivariateFunction() {
public double value(double x) {
if (x < lower) {
throw new NumberIsTooSmallException(x, lower, true);
} else if (x > upper) {
throw new NumberIsTooLargeException(x, upper, true);
} else {
return x;
}
}
};
UnivariateOptimizer optimizer = new BrentOptimizer(1e-10, 1e-14);
Assert.assertEquals(lower,
optimizer.optimize(100, f, GoalType.MINIMIZE, lower, upper).getPoint(),
1.0e-8);
Assert.assertEquals(upper,
optimizer.optimize(100, f, GoalType.MAXIMIZE, lower, upper).getPoint(),
1.0e-8);
}
@Test
public void testQuinticMin() {
// The function has local minima at -0.27195613 and 0.82221643.
UnivariateFunction f = new QuinticFunction();
UnivariateOptimizer optimizer = new BrentOptimizer(1e-10, 1e-14);
Assert.assertEquals(-0.27195613, optimizer.optimize(200, f, GoalType.MINIMIZE, -0.3, -0.2).getPoint(), 1.0e-8);
Assert.assertEquals( 0.82221643, optimizer.optimize(200, f, GoalType.MINIMIZE, 0.3, 0.9).getPoint(), 1.0e-8);
Assert.assertTrue(optimizer.getEvaluations() <= 50);
// search in a large interval
Assert.assertEquals(-0.27195613, optimizer.optimize(200, f, GoalType.MINIMIZE, -1.0, 0.2).getPoint(), 1.0e-8);
Assert.assertTrue(optimizer.getEvaluations() <= 50);
}
@Test
public void testQuinticMinStatistics() {
// The function has local minima at -0.27195613 and 0.82221643.
UnivariateFunction f = new QuinticFunction();
UnivariateOptimizer optimizer = new BrentOptimizer(1e-11, 1e-14);
final DescriptiveStatistics[] stat = new DescriptiveStatistics[2];
for (int i = 0; i < stat.length; i++) {
stat[i] = new DescriptiveStatistics();
}
final double min = -0.75;
final double max = 0.25;
final int nSamples = 200;
final double delta = (max - min) / nSamples;
for (int i = 0; i < nSamples; i++) {
final double start = min + i * delta;
stat[0].addValue(optimizer.optimize(40, f, GoalType.MINIMIZE, min, max, start).getPoint());
stat[1].addValue(optimizer.getEvaluations());
}
final double meanOptValue = stat[0].getMean();
final double medianEval = stat[1].getPercentile(50);
Assert.assertTrue(meanOptValue > -0.2719561281);
Assert.assertTrue(meanOptValue < -0.2719561280);
Assert.assertEquals(23, (int) medianEval);
}
@Test
public void testQuinticMax() {
// The quintic function has zeros at 0, +-0.5 and +-1.
// The function has a local maximum at 0.27195613.
UnivariateFunction f = new QuinticFunction();
UnivariateOptimizer optimizer = new BrentOptimizer(1e-12, 1e-14);
Assert.assertEquals(0.27195613, optimizer.optimize(100, f, GoalType.MAXIMIZE, 0.2, 0.3).getPoint(), 1e-8);
try {
optimizer.optimize(5, f, GoalType.MAXIMIZE, 0.2, 0.3);
Assert.fail("an exception should have been thrown");
} catch (TooManyEvaluationsException miee) {
// expected
}
}
@Test
public void testMinEndpoints() {
UnivariateFunction f = new Sin();
UnivariateOptimizer optimizer = new BrentOptimizer(1e-8, 1e-14);
// endpoint is minimum
double result = optimizer.optimize(50, f, GoalType.MINIMIZE, 3 * Math.PI / 2, 5).getPoint();
Assert.assertEquals(3 * Math.PI / 2, result, 1e-6);
result = optimizer.optimize(50, f, GoalType.MINIMIZE, 4, 3 * Math.PI / 2).getPoint();
Assert.assertEquals(3 * Math.PI / 2, result, 1e-6);
}
@Test
public void testMath832() {
final UnivariateFunction f = new UnivariateFunction() {
public double value(double x) {
final double sqrtX = FastMath.sqrt(x);
final double a = 1e2 * sqrtX;
final double b = 1e6 / x;
final double c = 1e4 / sqrtX;
return a + b + c;
}
};
UnivariateOptimizer optimizer = new BrentOptimizer(1e-10, 1e-8);
final double result = optimizer.optimize(1483,
f,
GoalType.MINIMIZE,
Double.MIN_VALUE,
Double.MAX_VALUE).getPoint();
Assert.assertEquals(804.9355825, result, 1e-6);
}
/**
* Contrived example showing that prior to the resolution of MATH-855
* (second revision), the algorithm would not return the best point if
* it happened to be the initial guess.
*/
@Test
public void testKeepInitIfBest() {
final double minSin = 3 * Math.PI / 2;
final double offset = 1e-8;
final double delta = 1e-7;
final UnivariateFunction f1 = new Sin();
final UnivariateFunction f2 = new StepFunction(new double[] { minSin, minSin + offset, minSin + 2 * offset},
new double[] { 0, -1, 0 });
final UnivariateFunction f = FunctionUtils.add(f1, f2);
// A slightly less stringent tolerance would make the test pass
// even with the previous implementation.
final double relTol = 1e-8;
final UnivariateOptimizer optimizer = new BrentOptimizer(relTol, 1e-100);
final double init = minSin + 1.5 * offset;
final UnivariatePointValuePair result
= optimizer.optimize(200, f, GoalType.MINIMIZE,
minSin - 6.789 * delta,
minSin + 9.876 * delta,
init);
final int numEval = optimizer.getEvaluations();
final double sol = result.getPoint();
final double expected = init;
// System.out.println("numEval=" + numEval);
// System.out.println("min=" + init + " f=" + f.value(init));
// System.out.println("sol=" + sol + " f=" + f.value(sol));
// System.out.println("exp=" + expected + " f=" + f.value(expected));
Assert.assertTrue("Best point not reported", f.value(sol) <= f.value(expected));
}
/**
* Contrived example showing that prior to the resolution of MATH-855,
* the algorithm, by always returning the last evaluated point, would
* sometimes not report the best point it had found.
*/
@Test
public void testMath855() {
final double minSin = 3 * Math.PI / 2;
final double offset = 1e-8;
final double delta = 1e-7;
final UnivariateFunction f1 = new Sin();
final UnivariateFunction f2 = new StepFunction(new double[] { minSin, minSin + offset, minSin + 5 * offset },
new double[] { 0, -1, 0 });
final UnivariateFunction f = FunctionUtils.add(f1, f2);
final UnivariateOptimizer optimizer = new BrentOptimizer(1e-8, 1e-100);
final UnivariatePointValuePair result
= optimizer.optimize(200, f, GoalType.MINIMIZE,
minSin - 6.789 * delta,
minSin + 9.876 * delta);
final int numEval = optimizer.getEvaluations();
final double sol = result.getPoint();
final double expected = 4.712389027602411;
// System.out.println("min=" + (minSin + offset) + " f=" + f.value(minSin + offset));
// System.out.println("sol=" + sol + " f=" + f.value(sol));
// System.out.println("exp=" + expected + " f=" + f.value(expected));
Assert.assertTrue("Best point not reported", f.value(sol) <= f.value(expected));
}
} | // You are a professional Java test case writer, please create a test case named `testKeepInitIfBest` for the issue `Math-MATH-855`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Math-MATH-855
//
// ## Issue-Title:
// "BrentOptimizer" not always reporting the best point
//
// ## Issue-Description:
//
// BrentOptimizer (package "o.a.c.m.optimization.univariate") does not check that the point it is going to return is indeed the best one it has encountered. Indeed, the last evaluated point might be slightly worse than the one before last.
//
//
//
//
//
@Test
public void testKeepInitIfBest() {
| 221 | /**
* Contrived example showing that prior to the resolution of MATH-855
* (second revision), the algorithm would not return the best point if
* it happened to be the initial guess.
*/ | 23 | 191 | src/test/java/org/apache/commons/math3/optimization/univariate/BrentOptimizerTest.java | src/test/java | ```markdown
## Issue-ID: Math-MATH-855
## Issue-Title:
"BrentOptimizer" not always reporting the best point
## Issue-Description:
BrentOptimizer (package "o.a.c.m.optimization.univariate") does not check that the point it is going to return is indeed the best one it has encountered. Indeed, the last evaluated point might be slightly worse than the one before last.
```
You are a professional Java test case writer, please create a test case named `testKeepInitIfBest` for the issue `Math-MATH-855`, utilizing the provided issue report information and the following function signature.
```java
@Test
public void testKeepInitIfBest() {
```
| 191 | [
"org.apache.commons.math3.optimization.univariate.BrentOptimizer"
] | 7fccde03d3c29ad6ef8a8ba68491dddf0ffa99fc027bbea066c5d38a129704b7 | @Test
public void testKeepInitIfBest() | // You are a professional Java test case writer, please create a test case named `testKeepInitIfBest` for the issue `Math-MATH-855`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Math-MATH-855
//
// ## Issue-Title:
// "BrentOptimizer" not always reporting the best point
//
// ## Issue-Description:
//
// BrentOptimizer (package "o.a.c.m.optimization.univariate") does not check that the point it is going to return is indeed the best one it has encountered. Indeed, the last evaluated point might be slightly worse than the one before last.
//
//
//
//
//
| Math | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.math3.optimization.univariate;
import org.apache.commons.math3.analysis.QuinticFunction;
import org.apache.commons.math3.analysis.UnivariateFunction;
import org.apache.commons.math3.analysis.function.Sin;
import org.apache.commons.math3.analysis.function.StepFunction;
import org.apache.commons.math3.analysis.FunctionUtils;
import org.apache.commons.math3.exception.NumberIsTooLargeException;
import org.apache.commons.math3.exception.NumberIsTooSmallException;
import org.apache.commons.math3.exception.TooManyEvaluationsException;
import org.apache.commons.math3.optimization.ConvergenceChecker;
import org.apache.commons.math3.optimization.GoalType;
import org.apache.commons.math3.stat.descriptive.DescriptiveStatistics;
import org.apache.commons.math3.util.FastMath;
import org.junit.Assert;
import org.junit.Test;
/**
* @version $Id$
*/
public final class BrentOptimizerTest {
@Test
public void testSinMin() {
UnivariateFunction f = new Sin();
UnivariateOptimizer optimizer = new BrentOptimizer(1e-10, 1e-14);
Assert.assertEquals(3 * Math.PI / 2, optimizer.optimize(200, f, GoalType.MINIMIZE, 4, 5).getPoint(), 1e-8);
Assert.assertTrue(optimizer.getEvaluations() <= 50);
Assert.assertEquals(200, optimizer.getMaxEvaluations());
Assert.assertEquals(3 * Math.PI / 2, optimizer.optimize(200, f, GoalType.MINIMIZE, 1, 5).getPoint(), 1e-8);
Assert.assertTrue(optimizer.getEvaluations() <= 100);
Assert.assertTrue(optimizer.getEvaluations() >= 15);
try {
optimizer.optimize(10, f, GoalType.MINIMIZE, 4, 5);
Assert.fail("an exception should have been thrown");
} catch (TooManyEvaluationsException fee) {
// expected
}
}
@Test
public void testSinMinWithValueChecker() {
final UnivariateFunction f = new Sin();
final ConvergenceChecker<UnivariatePointValuePair> checker = new SimpleUnivariateValueChecker(1e-5, 1e-14);
// The default stopping criterion of Brent's algorithm should not
// pass, but the search will stop at the given relative tolerance
// for the function value.
final UnivariateOptimizer optimizer = new BrentOptimizer(1e-10, 1e-14, checker);
final UnivariatePointValuePair result = optimizer.optimize(200, f, GoalType.MINIMIZE, 4, 5);
Assert.assertEquals(3 * Math.PI / 2, result.getPoint(), 1e-3);
}
@Test
public void testBoundaries() {
final double lower = -1.0;
final double upper = +1.0;
UnivariateFunction f = new UnivariateFunction() {
public double value(double x) {
if (x < lower) {
throw new NumberIsTooSmallException(x, lower, true);
} else if (x > upper) {
throw new NumberIsTooLargeException(x, upper, true);
} else {
return x;
}
}
};
UnivariateOptimizer optimizer = new BrentOptimizer(1e-10, 1e-14);
Assert.assertEquals(lower,
optimizer.optimize(100, f, GoalType.MINIMIZE, lower, upper).getPoint(),
1.0e-8);
Assert.assertEquals(upper,
optimizer.optimize(100, f, GoalType.MAXIMIZE, lower, upper).getPoint(),
1.0e-8);
}
@Test
public void testQuinticMin() {
// The function has local minima at -0.27195613 and 0.82221643.
UnivariateFunction f = new QuinticFunction();
UnivariateOptimizer optimizer = new BrentOptimizer(1e-10, 1e-14);
Assert.assertEquals(-0.27195613, optimizer.optimize(200, f, GoalType.MINIMIZE, -0.3, -0.2).getPoint(), 1.0e-8);
Assert.assertEquals( 0.82221643, optimizer.optimize(200, f, GoalType.MINIMIZE, 0.3, 0.9).getPoint(), 1.0e-8);
Assert.assertTrue(optimizer.getEvaluations() <= 50);
// search in a large interval
Assert.assertEquals(-0.27195613, optimizer.optimize(200, f, GoalType.MINIMIZE, -1.0, 0.2).getPoint(), 1.0e-8);
Assert.assertTrue(optimizer.getEvaluations() <= 50);
}
@Test
public void testQuinticMinStatistics() {
// The function has local minima at -0.27195613 and 0.82221643.
UnivariateFunction f = new QuinticFunction();
UnivariateOptimizer optimizer = new BrentOptimizer(1e-11, 1e-14);
final DescriptiveStatistics[] stat = new DescriptiveStatistics[2];
for (int i = 0; i < stat.length; i++) {
stat[i] = new DescriptiveStatistics();
}
final double min = -0.75;
final double max = 0.25;
final int nSamples = 200;
final double delta = (max - min) / nSamples;
for (int i = 0; i < nSamples; i++) {
final double start = min + i * delta;
stat[0].addValue(optimizer.optimize(40, f, GoalType.MINIMIZE, min, max, start).getPoint());
stat[1].addValue(optimizer.getEvaluations());
}
final double meanOptValue = stat[0].getMean();
final double medianEval = stat[1].getPercentile(50);
Assert.assertTrue(meanOptValue > -0.2719561281);
Assert.assertTrue(meanOptValue < -0.2719561280);
Assert.assertEquals(23, (int) medianEval);
}
@Test
public void testQuinticMax() {
// The quintic function has zeros at 0, +-0.5 and +-1.
// The function has a local maximum at 0.27195613.
UnivariateFunction f = new QuinticFunction();
UnivariateOptimizer optimizer = new BrentOptimizer(1e-12, 1e-14);
Assert.assertEquals(0.27195613, optimizer.optimize(100, f, GoalType.MAXIMIZE, 0.2, 0.3).getPoint(), 1e-8);
try {
optimizer.optimize(5, f, GoalType.MAXIMIZE, 0.2, 0.3);
Assert.fail("an exception should have been thrown");
} catch (TooManyEvaluationsException miee) {
// expected
}
}
@Test
public void testMinEndpoints() {
UnivariateFunction f = new Sin();
UnivariateOptimizer optimizer = new BrentOptimizer(1e-8, 1e-14);
// endpoint is minimum
double result = optimizer.optimize(50, f, GoalType.MINIMIZE, 3 * Math.PI / 2, 5).getPoint();
Assert.assertEquals(3 * Math.PI / 2, result, 1e-6);
result = optimizer.optimize(50, f, GoalType.MINIMIZE, 4, 3 * Math.PI / 2).getPoint();
Assert.assertEquals(3 * Math.PI / 2, result, 1e-6);
}
@Test
public void testMath832() {
final UnivariateFunction f = new UnivariateFunction() {
public double value(double x) {
final double sqrtX = FastMath.sqrt(x);
final double a = 1e2 * sqrtX;
final double b = 1e6 / x;
final double c = 1e4 / sqrtX;
return a + b + c;
}
};
UnivariateOptimizer optimizer = new BrentOptimizer(1e-10, 1e-8);
final double result = optimizer.optimize(1483,
f,
GoalType.MINIMIZE,
Double.MIN_VALUE,
Double.MAX_VALUE).getPoint();
Assert.assertEquals(804.9355825, result, 1e-6);
}
/**
* Contrived example showing that prior to the resolution of MATH-855
* (second revision), the algorithm would not return the best point if
* it happened to be the initial guess.
*/
@Test
public void testKeepInitIfBest() {
final double minSin = 3 * Math.PI / 2;
final double offset = 1e-8;
final double delta = 1e-7;
final UnivariateFunction f1 = new Sin();
final UnivariateFunction f2 = new StepFunction(new double[] { minSin, minSin + offset, minSin + 2 * offset},
new double[] { 0, -1, 0 });
final UnivariateFunction f = FunctionUtils.add(f1, f2);
// A slightly less stringent tolerance would make the test pass
// even with the previous implementation.
final double relTol = 1e-8;
final UnivariateOptimizer optimizer = new BrentOptimizer(relTol, 1e-100);
final double init = minSin + 1.5 * offset;
final UnivariatePointValuePair result
= optimizer.optimize(200, f, GoalType.MINIMIZE,
minSin - 6.789 * delta,
minSin + 9.876 * delta,
init);
final int numEval = optimizer.getEvaluations();
final double sol = result.getPoint();
final double expected = init;
// System.out.println("numEval=" + numEval);
// System.out.println("min=" + init + " f=" + f.value(init));
// System.out.println("sol=" + sol + " f=" + f.value(sol));
// System.out.println("exp=" + expected + " f=" + f.value(expected));
Assert.assertTrue("Best point not reported", f.value(sol) <= f.value(expected));
}
/**
* Contrived example showing that prior to the resolution of MATH-855,
* the algorithm, by always returning the last evaluated point, would
* sometimes not report the best point it had found.
*/
@Test
public void testMath855() {
final double minSin = 3 * Math.PI / 2;
final double offset = 1e-8;
final double delta = 1e-7;
final UnivariateFunction f1 = new Sin();
final UnivariateFunction f2 = new StepFunction(new double[] { minSin, minSin + offset, minSin + 5 * offset },
new double[] { 0, -1, 0 });
final UnivariateFunction f = FunctionUtils.add(f1, f2);
final UnivariateOptimizer optimizer = new BrentOptimizer(1e-8, 1e-100);
final UnivariatePointValuePair result
= optimizer.optimize(200, f, GoalType.MINIMIZE,
minSin - 6.789 * delta,
minSin + 9.876 * delta);
final int numEval = optimizer.getEvaluations();
final double sol = result.getPoint();
final double expected = 4.712389027602411;
// System.out.println("min=" + (minSin + offset) + " f=" + f.value(minSin + offset));
// System.out.println("sol=" + sol + " f=" + f.value(sol));
// System.out.println("exp=" + expected + " f=" + f.value(expected));
Assert.assertTrue("Best point not reported", f.value(sol) <= f.value(expected));
}
} |
|
public void testForID_String_old() {
Map<String, String> map = new LinkedHashMap<String, String>();
map.put("GMT", "UTC");
map.put("WET", "WET");
map.put("CET", "CET");
map.put("MET", "CET");
map.put("ECT", "CET");
map.put("EET", "EET");
map.put("MIT", "Pacific/Apia");
map.put("HST", "Pacific/Honolulu");
map.put("AST", "America/Anchorage");
map.put("PST", "America/Los_Angeles");
map.put("MST", "America/Denver");
map.put("PNT", "America/Phoenix");
map.put("CST", "America/Chicago");
map.put("EST", "America/New_York");
map.put("IET", "America/Indiana/Indianapolis");
map.put("PRT", "America/Puerto_Rico");
map.put("CNT", "America/St_Johns");
map.put("AGT", "America/Argentina/Buenos_Aires");
map.put("BET", "America/Sao_Paulo");
map.put("ART", "Africa/Cairo");
map.put("CAT", "Africa/Harare");
map.put("EAT", "Africa/Addis_Ababa");
map.put("NET", "Asia/Yerevan");
map.put("PLT", "Asia/Karachi");
map.put("IST", "Asia/Kolkata");
map.put("BST", "Asia/Dhaka");
map.put("VST", "Asia/Ho_Chi_Minh");
map.put("CTT", "Asia/Shanghai");
map.put("JST", "Asia/Tokyo");
map.put("ACT", "Australia/Darwin");
map.put("AET", "Australia/Sydney");
map.put("SST", "Pacific/Guadalcanal");
map.put("NST", "Pacific/Auckland");
for (String key : map.keySet()) {
String value = map.get(key);
TimeZone juZone = TimeZone.getTimeZone(key);
DateTimeZone zone = DateTimeZone.forTimeZone(juZone);
assertEquals(value, zone.getID());
// System.out.println(juZone);
// System.out.println(juZone.getDisplayName());
// System.out.println(zone);
// System.out.println("------");
}
} | org.joda.time.TestDateTimeZone::testForID_String_old | src/test/java/org/joda/time/TestDateTimeZone.java | 287 | src/test/java/org/joda/time/TestDateTimeZone.java | testForID_String_old | /*
* Copyright 2001-2006 Stephen Colebourne
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.joda.time;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.io.PrintStream;
import java.lang.reflect.Modifier;
import java.security.AllPermission;
import java.security.CodeSource;
import java.security.Permission;
import java.security.PermissionCollection;
import java.security.Permissions;
import java.security.Policy;
import java.security.ProtectionDomain;
import java.util.HashSet;
import java.util.LinkedHashMap;
import java.util.Locale;
import java.util.Map;
import java.util.Set;
import java.util.TimeZone;
import junit.framework.TestCase;
import junit.framework.TestSuite;
import org.joda.time.tz.DefaultNameProvider;
import org.joda.time.tz.NameProvider;
import org.joda.time.tz.Provider;
import org.joda.time.tz.UTCProvider;
import org.joda.time.tz.ZoneInfoProvider;
/**
* This class is a JUnit test for DateTimeZone.
*
* @author Stephen Colebourne
*/
public class TestDateTimeZone extends TestCase {
private static final boolean OLD_JDK;
static {
String str = System.getProperty("java.version");
boolean old = true;
if (str.length() > 3 &&
str.charAt(0) == '1' &&
str.charAt(1) == '.' &&
(str.charAt(2) == '4' || str.charAt(2) == '5' || str.charAt(2) == '6')) {
old = false;
}
OLD_JDK = old;
}
// Test in 2002/03 as time zones are more well known
// (before the late 90's they were all over the place)
private static final DateTimeZone PARIS = DateTimeZone.forID("Europe/Paris");
private static final DateTimeZone LONDON = DateTimeZone.forID("Europe/London");
long y2002days = 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 +
365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365;
long y2003days = 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 +
365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365 + 365;
// 2002-06-09
private long TEST_TIME_SUMMER =
(y2002days + 31L + 28L + 31L + 30L + 31L + 9L -1L) * DateTimeConstants.MILLIS_PER_DAY;
// 2002-01-09
private long TEST_TIME_WINTER =
(y2002days + 9L -1L) * DateTimeConstants.MILLIS_PER_DAY;
// // 2002-04-05 Fri
// private long TEST_TIME1 =
// (y2002days + 31L + 28L + 31L + 5L -1L) * DateTimeConstants.MILLIS_PER_DAY
// + 12L * DateTimeConstants.MILLIS_PER_HOUR
// + 24L * DateTimeConstants.MILLIS_PER_MINUTE;
//
// // 2003-05-06 Tue
// private long TEST_TIME2 =
// (y2003days + 31L + 28L + 31L + 30L + 6L -1L) * DateTimeConstants.MILLIS_PER_DAY
// + 14L * DateTimeConstants.MILLIS_PER_HOUR
// + 28L * DateTimeConstants.MILLIS_PER_MINUTE;
private static final Policy RESTRICT;
private static final Policy ALLOW;
static {
// don't call Policy.getPolicy()
RESTRICT = new Policy() {
public PermissionCollection getPermissions(CodeSource codesource) {
Permissions p = new Permissions();
p.add(new AllPermission()); // enable everything
return p;
}
public void refresh() {
}
public boolean implies(ProtectionDomain domain, Permission permission) {
if (permission instanceof JodaTimePermission) {
return false;
}
return true;
// return super.implies(domain, permission);
}
};
ALLOW = new Policy() {
public PermissionCollection getPermissions(CodeSource codesource) {
Permissions p = new Permissions();
p.add(new AllPermission()); // enable everything
return p;
}
public void refresh() {
}
};
}
private DateTimeZone zone;
private Locale locale;
public static void main(String[] args) {
junit.textui.TestRunner.run(suite());
}
public static TestSuite suite() {
return new TestSuite(TestDateTimeZone.class);
}
public TestDateTimeZone(String name) {
super(name);
}
protected void setUp() throws Exception {
locale = Locale.getDefault();
zone = DateTimeZone.getDefault();
Locale.setDefault(Locale.UK);
}
protected void tearDown() throws Exception {
Locale.setDefault(locale);
DateTimeZone.setDefault(zone);
}
//-----------------------------------------------------------------------
public void testDefault() {
assertNotNull(DateTimeZone.getDefault());
DateTimeZone.setDefault(PARIS);
assertSame(PARIS, DateTimeZone.getDefault());
try {
DateTimeZone.setDefault(null);
fail();
} catch (IllegalArgumentException ex) {}
}
public void testDefaultSecurity() {
if (OLD_JDK) {
return;
}
try {
Policy.setPolicy(RESTRICT);
System.setSecurityManager(new SecurityManager());
DateTimeZone.setDefault(PARIS);
fail();
} catch (SecurityException ex) {
// ok
} finally {
System.setSecurityManager(null);
Policy.setPolicy(ALLOW);
}
}
//-----------------------------------------------------------------------
public void testForID_String() {
assertEquals(DateTimeZone.getDefault(), DateTimeZone.forID((String) null));
DateTimeZone zone = DateTimeZone.forID("Europe/London");
assertEquals("Europe/London", zone.getID());
zone = DateTimeZone.forID("UTC");
assertSame(DateTimeZone.UTC, zone);
zone = DateTimeZone.forID("+00:00");
assertSame(DateTimeZone.UTC, zone);
zone = DateTimeZone.forID("+00");
assertSame(DateTimeZone.UTC, zone);
zone = DateTimeZone.forID("+01:23");
assertEquals("+01:23", zone.getID());
assertEquals(DateTimeConstants.MILLIS_PER_HOUR + (23L * DateTimeConstants.MILLIS_PER_MINUTE),
zone.getOffset(TEST_TIME_SUMMER));
zone = DateTimeZone.forID("-02:00");
assertEquals("-02:00", zone.getID());
assertEquals((-2L * DateTimeConstants.MILLIS_PER_HOUR),
zone.getOffset(TEST_TIME_SUMMER));
zone = DateTimeZone.forID("-07:05:34.0");
assertEquals("-07:05:34", zone.getID());
assertEquals((-7L * DateTimeConstants.MILLIS_PER_HOUR) +
(-5L * DateTimeConstants.MILLIS_PER_MINUTE) +
(-34L * DateTimeConstants.MILLIS_PER_SECOND),
zone.getOffset(TEST_TIME_SUMMER));
try {
DateTimeZone.forID("SST");
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.forID("europe/london");
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.forID("Europe/UK");
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.forID("+");
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.forID("+0");
fail();
} catch (IllegalArgumentException ex) {}
}
public void testForID_String_old() {
Map<String, String> map = new LinkedHashMap<String, String>();
map.put("GMT", "UTC");
map.put("WET", "WET");
map.put("CET", "CET");
map.put("MET", "CET");
map.put("ECT", "CET");
map.put("EET", "EET");
map.put("MIT", "Pacific/Apia");
map.put("HST", "Pacific/Honolulu");
map.put("AST", "America/Anchorage");
map.put("PST", "America/Los_Angeles");
map.put("MST", "America/Denver");
map.put("PNT", "America/Phoenix");
map.put("CST", "America/Chicago");
map.put("EST", "America/New_York");
map.put("IET", "America/Indiana/Indianapolis");
map.put("PRT", "America/Puerto_Rico");
map.put("CNT", "America/St_Johns");
map.put("AGT", "America/Argentina/Buenos_Aires");
map.put("BET", "America/Sao_Paulo");
map.put("ART", "Africa/Cairo");
map.put("CAT", "Africa/Harare");
map.put("EAT", "Africa/Addis_Ababa");
map.put("NET", "Asia/Yerevan");
map.put("PLT", "Asia/Karachi");
map.put("IST", "Asia/Kolkata");
map.put("BST", "Asia/Dhaka");
map.put("VST", "Asia/Ho_Chi_Minh");
map.put("CTT", "Asia/Shanghai");
map.put("JST", "Asia/Tokyo");
map.put("ACT", "Australia/Darwin");
map.put("AET", "Australia/Sydney");
map.put("SST", "Pacific/Guadalcanal");
map.put("NST", "Pacific/Auckland");
for (String key : map.keySet()) {
String value = map.get(key);
TimeZone juZone = TimeZone.getTimeZone(key);
DateTimeZone zone = DateTimeZone.forTimeZone(juZone);
assertEquals(value, zone.getID());
// System.out.println(juZone);
// System.out.println(juZone.getDisplayName());
// System.out.println(zone);
// System.out.println("------");
}
}
//-----------------------------------------------------------------------
public void testForOffsetHours_int() {
assertEquals(DateTimeZone.UTC, DateTimeZone.forOffsetHours(0));
assertEquals(DateTimeZone.forID("+03:00"), DateTimeZone.forOffsetHours(3));
assertEquals(DateTimeZone.forID("-02:00"), DateTimeZone.forOffsetHours(-2));
try {
DateTimeZone.forOffsetHours(999999);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testForOffsetHoursMinutes_int_int() {
assertEquals(DateTimeZone.UTC, DateTimeZone.forOffsetHoursMinutes(0, 0));
assertEquals(DateTimeZone.forID("+03:15"), DateTimeZone.forOffsetHoursMinutes(3, 15));
assertEquals(DateTimeZone.forID("-02:00"), DateTimeZone.forOffsetHoursMinutes(-2, 0));
assertEquals(DateTimeZone.forID("-02:30"), DateTimeZone.forOffsetHoursMinutes(-2, 30));
try {
DateTimeZone.forOffsetHoursMinutes(2, 60);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.forOffsetHoursMinutes(-2, 60);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.forOffsetHoursMinutes(2, -1);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.forOffsetHoursMinutes(-2, -1);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.forOffsetHoursMinutes(999999, 0);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testForOffsetMillis_int() {
assertSame(DateTimeZone.UTC, DateTimeZone.forOffsetMillis(0));
assertEquals(DateTimeZone.forID("+03:00"), DateTimeZone.forOffsetMillis(3 * 60 * 60 * 1000));
assertEquals(DateTimeZone.forID("-02:00"), DateTimeZone.forOffsetMillis(-2 * 60 * 60 * 1000));
assertEquals(DateTimeZone.forID("+04:45:17.045"),
DateTimeZone.forOffsetMillis(
4 * 60 * 60 * 1000 + 45 * 60 * 1000 + 17 * 1000 + 45));
}
//-----------------------------------------------------------------------
public void testForTimeZone_TimeZone() {
assertEquals(DateTimeZone.getDefault(), DateTimeZone.forTimeZone((TimeZone) null));
DateTimeZone zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("Europe/London"));
assertEquals("Europe/London", zone.getID());
assertSame(DateTimeZone.UTC, DateTimeZone.forTimeZone(TimeZone.getTimeZone("UTC")));
zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("+00:00"));
assertSame(DateTimeZone.UTC, zone);
zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("GMT+00:00"));
assertSame(DateTimeZone.UTC, zone);
zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("GMT+00:00"));
assertSame(DateTimeZone.UTC, zone);
zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("GMT+00"));
assertSame(DateTimeZone.UTC, zone);
zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("GMT+01:23"));
assertEquals("+01:23", zone.getID());
assertEquals(DateTimeConstants.MILLIS_PER_HOUR + (23L * DateTimeConstants.MILLIS_PER_MINUTE),
zone.getOffset(TEST_TIME_SUMMER));
zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("GMT-02:00"));
assertEquals("-02:00", zone.getID());
assertEquals((-2L * DateTimeConstants.MILLIS_PER_HOUR), zone.getOffset(TEST_TIME_SUMMER));
zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("EST"));
assertEquals("America/New_York", zone.getID());
}
public void testTimeZoneConversion() {
TimeZone jdkTimeZone = TimeZone.getTimeZone("GMT-10");
assertEquals("GMT-10:00", jdkTimeZone.getID());
DateTimeZone jodaTimeZone = DateTimeZone.forTimeZone(jdkTimeZone);
assertEquals("-10:00", jodaTimeZone.getID());
assertEquals(jdkTimeZone.getRawOffset(), jodaTimeZone.getOffset(0L));
TimeZone convertedTimeZone = jodaTimeZone.toTimeZone();
assertEquals("GMT-10:00", jdkTimeZone.getID());
assertEquals(jdkTimeZone.getID(), convertedTimeZone.getID());
assertEquals(jdkTimeZone.getRawOffset(), convertedTimeZone.getRawOffset());
}
//-----------------------------------------------------------------------
public void testGetAvailableIDs() {
assertTrue(DateTimeZone.getAvailableIDs().contains("UTC"));
}
//-----------------------------------------------------------------------
public void testProvider() {
try {
assertNotNull(DateTimeZone.getProvider());
Provider provider = DateTimeZone.getProvider();
DateTimeZone.setProvider(null);
assertEquals(provider.getClass(), DateTimeZone.getProvider().getClass());
try {
DateTimeZone.setProvider(new MockNullIDSProvider());
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.setProvider(new MockEmptyIDSProvider());
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.setProvider(new MockNoUTCProvider());
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.setProvider(new MockBadUTCProvider());
fail();
} catch (IllegalArgumentException ex) {}
Provider prov = new MockOKProvider();
DateTimeZone.setProvider(prov);
assertSame(prov, DateTimeZone.getProvider());
assertEquals(2, DateTimeZone.getAvailableIDs().size());
assertTrue(DateTimeZone.getAvailableIDs().contains("UTC"));
assertTrue(DateTimeZone.getAvailableIDs().contains("Europe/London"));
} finally {
DateTimeZone.setProvider(null);
assertEquals(ZoneInfoProvider.class, DateTimeZone.getProvider().getClass());
}
try {
System.setProperty("org.joda.time.DateTimeZone.Provider", "org.joda.time.tz.UTCProvider");
DateTimeZone.setProvider(null);
assertEquals(UTCProvider.class, DateTimeZone.getProvider().getClass());
} finally {
System.getProperties().remove("org.joda.time.DateTimeZone.Provider");
DateTimeZone.setProvider(null);
assertEquals(ZoneInfoProvider.class, DateTimeZone.getProvider().getClass());
}
PrintStream syserr = System.err;
try {
System.setProperty("org.joda.time.DateTimeZone.Provider", "xxx");
ByteArrayOutputStream baos = new ByteArrayOutputStream();
System.setErr(new PrintStream(baos));
DateTimeZone.setProvider(null);
assertEquals(ZoneInfoProvider.class, DateTimeZone.getProvider().getClass());
String str = new String(baos.toByteArray());
assertTrue(str.indexOf("java.lang.ClassNotFoundException") >= 0);
} finally {
System.setErr(syserr);
System.getProperties().remove("org.joda.time.DateTimeZone.Provider");
DateTimeZone.setProvider(null);
assertEquals(ZoneInfoProvider.class, DateTimeZone.getProvider().getClass());
}
}
public void testProviderSecurity() {
if (OLD_JDK) {
return;
}
try {
Policy.setPolicy(RESTRICT);
System.setSecurityManager(new SecurityManager());
DateTimeZone.setProvider(new MockOKProvider());
fail();
} catch (SecurityException ex) {
// ok
} finally {
System.setSecurityManager(null);
Policy.setPolicy(ALLOW);
}
}
static class MockNullIDSProvider implements Provider {
public Set getAvailableIDs() {
return null;
}
public DateTimeZone getZone(String id) {
return null;
}
}
static class MockEmptyIDSProvider implements Provider {
public Set getAvailableIDs() {
return new HashSet();
}
public DateTimeZone getZone(String id) {
return null;
}
}
static class MockNoUTCProvider implements Provider {
public Set getAvailableIDs() {
Set set = new HashSet();
set.add("Europe/London");
return set;
}
public DateTimeZone getZone(String id) {
return null;
}
}
static class MockBadUTCProvider implements Provider {
public Set getAvailableIDs() {
Set set = new HashSet();
set.add("UTC");
set.add("Europe/London");
return set;
}
public DateTimeZone getZone(String id) {
return null;
}
}
static class MockOKProvider implements Provider {
public Set getAvailableIDs() {
Set set = new HashSet();
set.add("UTC");
set.add("Europe/London");
return set;
}
public DateTimeZone getZone(String id) {
return DateTimeZone.UTC;
}
}
//-----------------------------------------------------------------------
public void testNameProvider() {
try {
assertNotNull(DateTimeZone.getNameProvider());
NameProvider provider = DateTimeZone.getNameProvider();
DateTimeZone.setNameProvider(null);
assertEquals(provider.getClass(), DateTimeZone.getNameProvider().getClass());
provider = new MockOKButNullNameProvider();
DateTimeZone.setNameProvider(provider);
assertSame(provider, DateTimeZone.getNameProvider());
assertEquals("+00:00", DateTimeZone.UTC.getShortName(TEST_TIME_SUMMER));
assertEquals("+00:00", DateTimeZone.UTC.getName(TEST_TIME_SUMMER));
} finally {
DateTimeZone.setNameProvider(null);
}
try {
System.setProperty("org.joda.time.DateTimeZone.NameProvider", "org.joda.time.tz.DefaultNameProvider");
DateTimeZone.setNameProvider(null);
assertEquals(DefaultNameProvider.class, DateTimeZone.getNameProvider().getClass());
} finally {
System.getProperties().remove("org.joda.time.DateTimeZone.NameProvider");
DateTimeZone.setNameProvider(null);
assertEquals(DefaultNameProvider.class, DateTimeZone.getNameProvider().getClass());
}
PrintStream syserr = System.err;
try {
System.setProperty("org.joda.time.DateTimeZone.NameProvider", "xxx");
ByteArrayOutputStream baos = new ByteArrayOutputStream();
System.setErr(new PrintStream(baos));
DateTimeZone.setNameProvider(null);
assertEquals(DefaultNameProvider.class, DateTimeZone.getNameProvider().getClass());
String str = new String(baos.toByteArray());
assertTrue(str.indexOf("java.lang.ClassNotFoundException") >= 0);
} finally {
System.setErr(syserr);
System.getProperties().remove("org.joda.time.DateTimeZone.NameProvider");
DateTimeZone.setNameProvider(null);
assertEquals(DefaultNameProvider.class, DateTimeZone.getNameProvider().getClass());
}
}
public void testNameProviderSecurity() {
if (OLD_JDK) {
return;
}
try {
Policy.setPolicy(RESTRICT);
System.setSecurityManager(new SecurityManager());
DateTimeZone.setNameProvider(new MockOKButNullNameProvider());
fail();
} catch (SecurityException ex) {
// ok
} finally {
System.setSecurityManager(null);
Policy.setPolicy(ALLOW);
}
}
static class MockOKButNullNameProvider implements NameProvider {
public String getShortName(Locale locale, String id, String nameKey) {
return null;
}
public String getName(Locale locale, String id, String nameKey) {
return null;
}
}
//-----------------------------------------------------------------------
public void testConstructor() {
assertEquals(1, DateTimeZone.class.getDeclaredConstructors().length);
assertTrue(Modifier.isProtected(DateTimeZone.class.getDeclaredConstructors()[0].getModifiers()));
try {
new DateTimeZone(null) {
public String getNameKey(long instant) {
return null;
}
public int getOffset(long instant) {
return 0;
}
public int getStandardOffset(long instant) {
return 0;
}
public boolean isFixed() {
return false;
}
public long nextTransition(long instant) {
return 0;
}
public long previousTransition(long instant) {
return 0;
}
public boolean equals(Object object) {
return false;
}
};
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testGetID() {
DateTimeZone zone = DateTimeZone.forID("Europe/Paris");
assertEquals("Europe/Paris", zone.getID());
}
public void testGetNameKey() {
DateTimeZone zone = DateTimeZone.forID("Europe/London");
assertEquals("BST", zone.getNameKey(TEST_TIME_SUMMER));
assertEquals("GMT", zone.getNameKey(TEST_TIME_WINTER));
}
public void testGetShortName() {}
// Defects4J: flaky method
// public void testGetShortName() {
// DateTimeZone zone = DateTimeZone.forID("Europe/London");
// assertEquals("BST", zone.getShortName(TEST_TIME_SUMMER));
// assertEquals("GMT", zone.getShortName(TEST_TIME_WINTER));
// assertEquals("BST", zone.getShortName(TEST_TIME_SUMMER, Locale.ENGLISH));
// }
public void testGetShortNameProviderName() {
assertEquals(null, DateTimeZone.getNameProvider().getShortName(null, "Europe/London", "BST"));
assertEquals(null, DateTimeZone.getNameProvider().getShortName(Locale.ENGLISH, null, "BST"));
assertEquals(null, DateTimeZone.getNameProvider().getShortName(Locale.ENGLISH, "Europe/London", null));
assertEquals(null, DateTimeZone.getNameProvider().getShortName(null, null, null));
}
public void testGetShortNameNullKey() {
DateTimeZone zone = new MockDateTimeZone("Europe/London");
assertEquals("Europe/London", zone.getShortName(TEST_TIME_SUMMER, Locale.ENGLISH));
}
public void testGetName() {}
// Defects4J: flaky method
// public void testGetName() {
// DateTimeZone zone = DateTimeZone.forID("Europe/London");
// assertEquals("British Summer Time", zone.getName(TEST_TIME_SUMMER));
// assertEquals("Greenwich Mean Time", zone.getName(TEST_TIME_WINTER));
// assertEquals("British Summer Time", zone.getName(TEST_TIME_SUMMER, Locale.ENGLISH));
//
// }
public void testGetNameProviderName() {
assertEquals(null, DateTimeZone.getNameProvider().getName(null, "Europe/London", "BST"));
assertEquals(null, DateTimeZone.getNameProvider().getName(Locale.ENGLISH, null, "BST"));
assertEquals(null, DateTimeZone.getNameProvider().getName(Locale.ENGLISH, "Europe/London", null));
assertEquals(null, DateTimeZone.getNameProvider().getName(null, null, null));
}
public void testGetNameNullKey() {
DateTimeZone zone = new MockDateTimeZone("Europe/London");
assertEquals("Europe/London", zone.getName(TEST_TIME_SUMMER, Locale.ENGLISH));
}
static class MockDateTimeZone extends DateTimeZone {
public MockDateTimeZone(String id) {
super(id);
}
public String getNameKey(long instant) {
return null; // null
}
public int getOffset(long instant) {
return 0;
}
public int getStandardOffset(long instant) {
return 0;
}
public boolean isFixed() {
return false;
}
public long nextTransition(long instant) {
return 0;
}
public long previousTransition(long instant) {
return 0;
}
public boolean equals(Object object) {
return false;
}
}
//-----------------------------------------------------------------------
public void testGetOffset_long() {
DateTimeZone zone = DateTimeZone.forID("Europe/Paris");
assertEquals(2L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffset(TEST_TIME_SUMMER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffset(TEST_TIME_WINTER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getStandardOffset(TEST_TIME_SUMMER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getStandardOffset(TEST_TIME_WINTER));
assertEquals(2L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffsetFromLocal(TEST_TIME_SUMMER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffsetFromLocal(TEST_TIME_WINTER));
assertEquals(false, zone.isStandardOffset(TEST_TIME_SUMMER));
assertEquals(true, zone.isStandardOffset(TEST_TIME_WINTER));
}
public void testGetOffset_RI() {
DateTimeZone zone = DateTimeZone.forID("Europe/Paris");
assertEquals(2L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffset(new Instant(TEST_TIME_SUMMER)));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffset(new Instant(TEST_TIME_WINTER)));
assertEquals(zone.getOffset(DateTimeUtils.currentTimeMillis()), zone.getOffset(null));
}
public void testGetOffsetFixed() {
DateTimeZone zone = DateTimeZone.forID("+01:00");
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffset(TEST_TIME_SUMMER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffset(TEST_TIME_WINTER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getStandardOffset(TEST_TIME_SUMMER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getStandardOffset(TEST_TIME_WINTER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffsetFromLocal(TEST_TIME_SUMMER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffsetFromLocal(TEST_TIME_WINTER));
assertEquals(true, zone.isStandardOffset(TEST_TIME_SUMMER));
assertEquals(true, zone.isStandardOffset(TEST_TIME_WINTER));
}
public void testGetOffsetFixed_RI() {
DateTimeZone zone = DateTimeZone.forID("+01:00");
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffset(new Instant(TEST_TIME_SUMMER)));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffset(new Instant(TEST_TIME_WINTER)));
assertEquals(zone.getOffset(DateTimeUtils.currentTimeMillis()), zone.getOffset(null));
}
//-----------------------------------------------------------------------
public void testGetMillisKeepLocal() {
long millisLondon = TEST_TIME_SUMMER;
long millisParis = TEST_TIME_SUMMER - 1L * DateTimeConstants.MILLIS_PER_HOUR;
assertEquals(millisLondon, LONDON.getMillisKeepLocal(LONDON, millisLondon));
assertEquals(millisParis, LONDON.getMillisKeepLocal(LONDON, millisParis));
assertEquals(millisLondon, PARIS.getMillisKeepLocal(PARIS, millisLondon));
assertEquals(millisParis, PARIS.getMillisKeepLocal(PARIS, millisParis));
assertEquals(millisParis, LONDON.getMillisKeepLocal(PARIS, millisLondon));
assertEquals(millisLondon, PARIS.getMillisKeepLocal(LONDON, millisParis));
DateTimeZone zone = DateTimeZone.getDefault();
try {
DateTimeZone.setDefault(LONDON);
assertEquals(millisLondon, PARIS.getMillisKeepLocal(null, millisParis));
} finally {
DateTimeZone.setDefault(zone);
}
}
//-----------------------------------------------------------------------
public void testIsFixed() {
DateTimeZone zone = DateTimeZone.forID("Europe/Paris");
assertEquals(false, zone.isFixed());
assertEquals(true, DateTimeZone.UTC.isFixed());
}
//-----------------------------------------------------------------------
public void testTransitionFixed() {
DateTimeZone zone = DateTimeZone.forID("+01:00");
assertEquals(TEST_TIME_SUMMER, zone.nextTransition(TEST_TIME_SUMMER));
assertEquals(TEST_TIME_WINTER, zone.nextTransition(TEST_TIME_WINTER));
assertEquals(TEST_TIME_SUMMER, zone.previousTransition(TEST_TIME_SUMMER));
assertEquals(TEST_TIME_WINTER, zone.previousTransition(TEST_TIME_WINTER));
}
// //-----------------------------------------------------------------------
// public void testIsLocalDateTimeOverlap_Berlin() {
// DateTimeZone zone = DateTimeZone.forID("Europe/Berlin");
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 10, 28, 1, 0)));
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 10, 28, 1, 59, 59, 99)));
// assertEquals(true, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 10, 28, 2, 0)));
// assertEquals(true, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 10, 28, 2, 30)));
// assertEquals(true, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 10, 28, 2, 59, 59, 99)));
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 10, 28, 3, 0)));
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 10, 28, 4, 0)));
//
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 3, 25, 1, 30))); // before gap
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 3, 25, 2, 30))); // gap
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 3, 25, 3, 30))); // after gap
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 12, 24, 12, 34)));
// }
//
// //-----------------------------------------------------------------------
// public void testIsLocalDateTimeOverlap_NewYork() {
// DateTimeZone zone = DateTimeZone.forID("America/New_York");
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 11, 4, 0, 0)));
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 11, 4, 0, 59, 59, 99)));
// assertEquals(true, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 11, 4, 1, 0)));
// assertEquals(true, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 11, 4, 1, 30)));
// assertEquals(true, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 11, 4, 1, 59, 59, 99)));
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 11, 4, 2, 0)));
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 11, 4, 3, 0)));
//
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 3, 11, 1, 30))); // before gap
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 3, 11, 2, 30))); // gap
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 3, 11, 3, 30))); // after gap
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 12, 24, 12, 34)));
// }
//-----------------------------------------------------------------------
public void testIsLocalDateTimeGap_Berlin() {
DateTimeZone zone = DateTimeZone.forID("Europe/Berlin");
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 25, 1, 0)));
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 25, 1, 59, 59, 99)));
assertEquals(true, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 25, 2, 0)));
assertEquals(true, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 25, 2, 30)));
assertEquals(true, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 25, 2, 59, 59, 99)));
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 25, 3, 0)));
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 25, 4, 0)));
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 10, 28, 1, 30))); // before overlap
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 10, 28, 2, 30))); // overlap
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 10, 28, 3, 30))); // after overlap
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 12, 24, 12, 34)));
}
//-----------------------------------------------------------------------
public void testIsLocalDateTimeGap_NewYork() {
DateTimeZone zone = DateTimeZone.forID("America/New_York");
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 11, 1, 0)));
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 11, 1, 59, 59, 99)));
assertEquals(true, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 11, 2, 0)));
assertEquals(true, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 11, 2, 30)));
assertEquals(true, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 11, 2, 59, 59, 99)));
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 11, 3, 0)));
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 11, 4, 0)));
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 11, 4, 0, 30))); // before overlap
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 11, 4, 1, 30))); // overlap
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 11, 4, 2, 30))); // after overlap
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 12, 24, 12, 34)));
}
//-----------------------------------------------------------------------
public void testToTimeZone() {
DateTimeZone zone = DateTimeZone.forID("Europe/Paris");
TimeZone tz = zone.toTimeZone();
assertEquals("Europe/Paris", tz.getID());
}
//-----------------------------------------------------------------------
public void testEqualsHashCode() {
DateTimeZone zone1 = DateTimeZone.forID("Europe/Paris");
DateTimeZone zone2 = DateTimeZone.forID("Europe/Paris");
assertEquals(true, zone1.equals(zone1));
assertEquals(true, zone1.equals(zone2));
assertEquals(true, zone2.equals(zone1));
assertEquals(true, zone2.equals(zone2));
assertEquals(true, zone1.hashCode() == zone2.hashCode());
DateTimeZone zone3 = DateTimeZone.forID("Europe/London");
assertEquals(true, zone3.equals(zone3));
assertEquals(false, zone1.equals(zone3));
assertEquals(false, zone2.equals(zone3));
assertEquals(false, zone3.equals(zone1));
assertEquals(false, zone3.equals(zone2));
assertEquals(false, zone1.hashCode() == zone3.hashCode());
assertEquals(true, zone3.hashCode() == zone3.hashCode());
DateTimeZone zone4 = DateTimeZone.forID("+01:00");
assertEquals(true, zone4.equals(zone4));
assertEquals(false, zone1.equals(zone4));
assertEquals(false, zone2.equals(zone4));
assertEquals(false, zone3.equals(zone4));
assertEquals(false, zone4.equals(zone1));
assertEquals(false, zone4.equals(zone2));
assertEquals(false, zone4.equals(zone3));
assertEquals(false, zone1.hashCode() == zone4.hashCode());
assertEquals(true, zone4.hashCode() == zone4.hashCode());
DateTimeZone zone5 = DateTimeZone.forID("+02:00");
assertEquals(true, zone5.equals(zone5));
assertEquals(false, zone1.equals(zone5));
assertEquals(false, zone2.equals(zone5));
assertEquals(false, zone3.equals(zone5));
assertEquals(false, zone4.equals(zone5));
assertEquals(false, zone5.equals(zone1));
assertEquals(false, zone5.equals(zone2));
assertEquals(false, zone5.equals(zone3));
assertEquals(false, zone5.equals(zone4));
assertEquals(false, zone1.hashCode() == zone5.hashCode());
assertEquals(true, zone5.hashCode() == zone5.hashCode());
}
//-----------------------------------------------------------------------
public void testToString() {
DateTimeZone zone = DateTimeZone.forID("Europe/Paris");
assertEquals("Europe/Paris", zone.toString());
assertEquals("UTC", DateTimeZone.UTC.toString());
}
//-----------------------------------------------------------------------
public void testSerialization1() throws Exception {
DateTimeZone zone = DateTimeZone.forID("Europe/Paris");
ByteArrayOutputStream baos = new ByteArrayOutputStream();
ObjectOutputStream oos = new ObjectOutputStream(baos);
oos.writeObject(zone);
byte[] bytes = baos.toByteArray();
oos.close();
ByteArrayInputStream bais = new ByteArrayInputStream(bytes);
ObjectInputStream ois = new ObjectInputStream(bais);
DateTimeZone result = (DateTimeZone) ois.readObject();
ois.close();
assertSame(zone, result);
}
//-----------------------------------------------------------------------
public void testSerialization2() throws Exception {
DateTimeZone zone = DateTimeZone.forID("+01:00");
ByteArrayOutputStream baos = new ByteArrayOutputStream();
ObjectOutputStream oos = new ObjectOutputStream(baos);
oos.writeObject(zone);
byte[] bytes = baos.toByteArray();
oos.close();
ByteArrayInputStream bais = new ByteArrayInputStream(bytes);
ObjectInputStream ois = new ObjectInputStream(bais);
DateTimeZone result = (DateTimeZone) ois.readObject();
ois.close();
assertSame(zone, result);
}
public void testCommentParse() throws Exception {
// A bug in ZoneInfoCompiler's handling of comments broke Europe/Athens
// after 1980. This test is included to make sure it doesn't break again.
DateTimeZone zone = DateTimeZone.forID("Europe/Athens");
DateTime dt = new DateTime(2005, 5, 5, 20, 10, 15, 0, zone);
assertEquals(1115313015000L, dt.getMillis());
}
public void testPatchedNameKeysLondon() throws Exception {
// the tz database does not have unique name keys [1716305]
DateTimeZone zone = DateTimeZone.forID("Europe/London");
DateTime now = new DateTime(2007, 1, 1, 0, 0, 0, 0);
String str1 = zone.getName(now.getMillis());
String str2 = zone.getName(now.plusMonths(6).getMillis());
assertEquals(false, str1.equals(str2));
}
public void testPatchedNameKeysSydney() throws Exception {
// the tz database does not have unique name keys [1716305]
DateTimeZone zone = DateTimeZone.forID("Australia/Sydney");
DateTime now = new DateTime(2007, 1, 1, 0, 0, 0, 0);
String str1 = zone.getName(now.getMillis());
String str2 = zone.getName(now.plusMonths(6).getMillis());
assertEquals(false, str1.equals(str2));
}
public void testPatchedNameKeysSydneyHistoric() throws Exception {
// the tz database does not have unique name keys [1716305]
DateTimeZone zone = DateTimeZone.forID("Australia/Sydney");
DateTime now = new DateTime(1996, 1, 1, 0, 0, 0, 0);
String str1 = zone.getName(now.getMillis());
String str2 = zone.getName(now.plusMonths(6).getMillis());
assertEquals(false, str1.equals(str2));
}
public void testPatchedNameKeysGazaHistoric() throws Exception {
// the tz database does not have unique name keys [1716305]
DateTimeZone zone = DateTimeZone.forID("Africa/Johannesburg");
DateTime now = new DateTime(1943, 1, 1, 0, 0, 0, 0);
String str1 = zone.getName(now.getMillis());
String str2 = zone.getName(now.plusMonths(6).getMillis());
assertEquals(false, str1.equals(str2));
}
} | // You are a professional Java test case writer, please create a test case named `testForID_String_old` for the issue `Time-112`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Time-112
//
// ## Issue-Title:
// #112 Incorrect mapping of the MET time zone
//
//
//
//
//
// ## Issue-Description:
// This timezone is mapped to Asia/Tehran in DateTimeZone. It should be middle europena time.
//
//
// I know that this bug has been raised before (Incorrect mapping of the MET time zone - ID: 2012274), and there is a comment stating that you won't break backward compatibility to fix this bug.
//
//
// 1. I disagree that this is a backward compatibility argument
// 2. No matter how you look at it, it is a bug.
//
//
// You could very well state that ALL bugs won't be fixed, because of backward compatibility.
//
//
// I request again that this bug be fixed.
//
//
//
//
public void testForID_String_old() {
| 287 | 23 | 242 | src/test/java/org/joda/time/TestDateTimeZone.java | src/test/java | ```markdown
## Issue-ID: Time-112
## Issue-Title:
#112 Incorrect mapping of the MET time zone
## Issue-Description:
This timezone is mapped to Asia/Tehran in DateTimeZone. It should be middle europena time.
I know that this bug has been raised before (Incorrect mapping of the MET time zone - ID: 2012274), and there is a comment stating that you won't break backward compatibility to fix this bug.
1. I disagree that this is a backward compatibility argument
2. No matter how you look at it, it is a bug.
You could very well state that ALL bugs won't be fixed, because of backward compatibility.
I request again that this bug be fixed.
```
You are a professional Java test case writer, please create a test case named `testForID_String_old` for the issue `Time-112`, utilizing the provided issue report information and the following function signature.
```java
public void testForID_String_old() {
```
| 242 | [
"org.joda.time.DateTimeZone"
] | 801239f9e0aabc047ecc25f3b96dccf4a27a38e8bcec47ca5723ad186cdb7727 | public void testForID_String_old() | // You are a professional Java test case writer, please create a test case named `testForID_String_old` for the issue `Time-112`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Time-112
//
// ## Issue-Title:
// #112 Incorrect mapping of the MET time zone
//
//
//
//
//
// ## Issue-Description:
// This timezone is mapped to Asia/Tehran in DateTimeZone. It should be middle europena time.
//
//
// I know that this bug has been raised before (Incorrect mapping of the MET time zone - ID: 2012274), and there is a comment stating that you won't break backward compatibility to fix this bug.
//
//
// 1. I disagree that this is a backward compatibility argument
// 2. No matter how you look at it, it is a bug.
//
//
// You could very well state that ALL bugs won't be fixed, because of backward compatibility.
//
//
// I request again that this bug be fixed.
//
//
//
//
| Time | /*
* Copyright 2001-2006 Stephen Colebourne
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.joda.time;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.io.PrintStream;
import java.lang.reflect.Modifier;
import java.security.AllPermission;
import java.security.CodeSource;
import java.security.Permission;
import java.security.PermissionCollection;
import java.security.Permissions;
import java.security.Policy;
import java.security.ProtectionDomain;
import java.util.HashSet;
import java.util.LinkedHashMap;
import java.util.Locale;
import java.util.Map;
import java.util.Set;
import java.util.TimeZone;
import junit.framework.TestCase;
import junit.framework.TestSuite;
import org.joda.time.tz.DefaultNameProvider;
import org.joda.time.tz.NameProvider;
import org.joda.time.tz.Provider;
import org.joda.time.tz.UTCProvider;
import org.joda.time.tz.ZoneInfoProvider;
/**
* This class is a JUnit test for DateTimeZone.
*
* @author Stephen Colebourne
*/
public class TestDateTimeZone extends TestCase {
private static final boolean OLD_JDK;
static {
String str = System.getProperty("java.version");
boolean old = true;
if (str.length() > 3 &&
str.charAt(0) == '1' &&
str.charAt(1) == '.' &&
(str.charAt(2) == '4' || str.charAt(2) == '5' || str.charAt(2) == '6')) {
old = false;
}
OLD_JDK = old;
}
// Test in 2002/03 as time zones are more well known
// (before the late 90's they were all over the place)
private static final DateTimeZone PARIS = DateTimeZone.forID("Europe/Paris");
private static final DateTimeZone LONDON = DateTimeZone.forID("Europe/London");
long y2002days = 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 +
365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365;
long y2003days = 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 +
365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365 + 365;
// 2002-06-09
private long TEST_TIME_SUMMER =
(y2002days + 31L + 28L + 31L + 30L + 31L + 9L -1L) * DateTimeConstants.MILLIS_PER_DAY;
// 2002-01-09
private long TEST_TIME_WINTER =
(y2002days + 9L -1L) * DateTimeConstants.MILLIS_PER_DAY;
// // 2002-04-05 Fri
// private long TEST_TIME1 =
// (y2002days + 31L + 28L + 31L + 5L -1L) * DateTimeConstants.MILLIS_PER_DAY
// + 12L * DateTimeConstants.MILLIS_PER_HOUR
// + 24L * DateTimeConstants.MILLIS_PER_MINUTE;
//
// // 2003-05-06 Tue
// private long TEST_TIME2 =
// (y2003days + 31L + 28L + 31L + 30L + 6L -1L) * DateTimeConstants.MILLIS_PER_DAY
// + 14L * DateTimeConstants.MILLIS_PER_HOUR
// + 28L * DateTimeConstants.MILLIS_PER_MINUTE;
private static final Policy RESTRICT;
private static final Policy ALLOW;
static {
// don't call Policy.getPolicy()
RESTRICT = new Policy() {
public PermissionCollection getPermissions(CodeSource codesource) {
Permissions p = new Permissions();
p.add(new AllPermission()); // enable everything
return p;
}
public void refresh() {
}
public boolean implies(ProtectionDomain domain, Permission permission) {
if (permission instanceof JodaTimePermission) {
return false;
}
return true;
// return super.implies(domain, permission);
}
};
ALLOW = new Policy() {
public PermissionCollection getPermissions(CodeSource codesource) {
Permissions p = new Permissions();
p.add(new AllPermission()); // enable everything
return p;
}
public void refresh() {
}
};
}
private DateTimeZone zone;
private Locale locale;
public static void main(String[] args) {
junit.textui.TestRunner.run(suite());
}
public static TestSuite suite() {
return new TestSuite(TestDateTimeZone.class);
}
public TestDateTimeZone(String name) {
super(name);
}
protected void setUp() throws Exception {
locale = Locale.getDefault();
zone = DateTimeZone.getDefault();
Locale.setDefault(Locale.UK);
}
protected void tearDown() throws Exception {
Locale.setDefault(locale);
DateTimeZone.setDefault(zone);
}
//-----------------------------------------------------------------------
public void testDefault() {
assertNotNull(DateTimeZone.getDefault());
DateTimeZone.setDefault(PARIS);
assertSame(PARIS, DateTimeZone.getDefault());
try {
DateTimeZone.setDefault(null);
fail();
} catch (IllegalArgumentException ex) {}
}
public void testDefaultSecurity() {
if (OLD_JDK) {
return;
}
try {
Policy.setPolicy(RESTRICT);
System.setSecurityManager(new SecurityManager());
DateTimeZone.setDefault(PARIS);
fail();
} catch (SecurityException ex) {
// ok
} finally {
System.setSecurityManager(null);
Policy.setPolicy(ALLOW);
}
}
//-----------------------------------------------------------------------
public void testForID_String() {
assertEquals(DateTimeZone.getDefault(), DateTimeZone.forID((String) null));
DateTimeZone zone = DateTimeZone.forID("Europe/London");
assertEquals("Europe/London", zone.getID());
zone = DateTimeZone.forID("UTC");
assertSame(DateTimeZone.UTC, zone);
zone = DateTimeZone.forID("+00:00");
assertSame(DateTimeZone.UTC, zone);
zone = DateTimeZone.forID("+00");
assertSame(DateTimeZone.UTC, zone);
zone = DateTimeZone.forID("+01:23");
assertEquals("+01:23", zone.getID());
assertEquals(DateTimeConstants.MILLIS_PER_HOUR + (23L * DateTimeConstants.MILLIS_PER_MINUTE),
zone.getOffset(TEST_TIME_SUMMER));
zone = DateTimeZone.forID("-02:00");
assertEquals("-02:00", zone.getID());
assertEquals((-2L * DateTimeConstants.MILLIS_PER_HOUR),
zone.getOffset(TEST_TIME_SUMMER));
zone = DateTimeZone.forID("-07:05:34.0");
assertEquals("-07:05:34", zone.getID());
assertEquals((-7L * DateTimeConstants.MILLIS_PER_HOUR) +
(-5L * DateTimeConstants.MILLIS_PER_MINUTE) +
(-34L * DateTimeConstants.MILLIS_PER_SECOND),
zone.getOffset(TEST_TIME_SUMMER));
try {
DateTimeZone.forID("SST");
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.forID("europe/london");
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.forID("Europe/UK");
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.forID("+");
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.forID("+0");
fail();
} catch (IllegalArgumentException ex) {}
}
public void testForID_String_old() {
Map<String, String> map = new LinkedHashMap<String, String>();
map.put("GMT", "UTC");
map.put("WET", "WET");
map.put("CET", "CET");
map.put("MET", "CET");
map.put("ECT", "CET");
map.put("EET", "EET");
map.put("MIT", "Pacific/Apia");
map.put("HST", "Pacific/Honolulu");
map.put("AST", "America/Anchorage");
map.put("PST", "America/Los_Angeles");
map.put("MST", "America/Denver");
map.put("PNT", "America/Phoenix");
map.put("CST", "America/Chicago");
map.put("EST", "America/New_York");
map.put("IET", "America/Indiana/Indianapolis");
map.put("PRT", "America/Puerto_Rico");
map.put("CNT", "America/St_Johns");
map.put("AGT", "America/Argentina/Buenos_Aires");
map.put("BET", "America/Sao_Paulo");
map.put("ART", "Africa/Cairo");
map.put("CAT", "Africa/Harare");
map.put("EAT", "Africa/Addis_Ababa");
map.put("NET", "Asia/Yerevan");
map.put("PLT", "Asia/Karachi");
map.put("IST", "Asia/Kolkata");
map.put("BST", "Asia/Dhaka");
map.put("VST", "Asia/Ho_Chi_Minh");
map.put("CTT", "Asia/Shanghai");
map.put("JST", "Asia/Tokyo");
map.put("ACT", "Australia/Darwin");
map.put("AET", "Australia/Sydney");
map.put("SST", "Pacific/Guadalcanal");
map.put("NST", "Pacific/Auckland");
for (String key : map.keySet()) {
String value = map.get(key);
TimeZone juZone = TimeZone.getTimeZone(key);
DateTimeZone zone = DateTimeZone.forTimeZone(juZone);
assertEquals(value, zone.getID());
// System.out.println(juZone);
// System.out.println(juZone.getDisplayName());
// System.out.println(zone);
// System.out.println("------");
}
}
//-----------------------------------------------------------------------
public void testForOffsetHours_int() {
assertEquals(DateTimeZone.UTC, DateTimeZone.forOffsetHours(0));
assertEquals(DateTimeZone.forID("+03:00"), DateTimeZone.forOffsetHours(3));
assertEquals(DateTimeZone.forID("-02:00"), DateTimeZone.forOffsetHours(-2));
try {
DateTimeZone.forOffsetHours(999999);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testForOffsetHoursMinutes_int_int() {
assertEquals(DateTimeZone.UTC, DateTimeZone.forOffsetHoursMinutes(0, 0));
assertEquals(DateTimeZone.forID("+03:15"), DateTimeZone.forOffsetHoursMinutes(3, 15));
assertEquals(DateTimeZone.forID("-02:00"), DateTimeZone.forOffsetHoursMinutes(-2, 0));
assertEquals(DateTimeZone.forID("-02:30"), DateTimeZone.forOffsetHoursMinutes(-2, 30));
try {
DateTimeZone.forOffsetHoursMinutes(2, 60);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.forOffsetHoursMinutes(-2, 60);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.forOffsetHoursMinutes(2, -1);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.forOffsetHoursMinutes(-2, -1);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.forOffsetHoursMinutes(999999, 0);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testForOffsetMillis_int() {
assertSame(DateTimeZone.UTC, DateTimeZone.forOffsetMillis(0));
assertEquals(DateTimeZone.forID("+03:00"), DateTimeZone.forOffsetMillis(3 * 60 * 60 * 1000));
assertEquals(DateTimeZone.forID("-02:00"), DateTimeZone.forOffsetMillis(-2 * 60 * 60 * 1000));
assertEquals(DateTimeZone.forID("+04:45:17.045"),
DateTimeZone.forOffsetMillis(
4 * 60 * 60 * 1000 + 45 * 60 * 1000 + 17 * 1000 + 45));
}
//-----------------------------------------------------------------------
public void testForTimeZone_TimeZone() {
assertEquals(DateTimeZone.getDefault(), DateTimeZone.forTimeZone((TimeZone) null));
DateTimeZone zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("Europe/London"));
assertEquals("Europe/London", zone.getID());
assertSame(DateTimeZone.UTC, DateTimeZone.forTimeZone(TimeZone.getTimeZone("UTC")));
zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("+00:00"));
assertSame(DateTimeZone.UTC, zone);
zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("GMT+00:00"));
assertSame(DateTimeZone.UTC, zone);
zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("GMT+00:00"));
assertSame(DateTimeZone.UTC, zone);
zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("GMT+00"));
assertSame(DateTimeZone.UTC, zone);
zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("GMT+01:23"));
assertEquals("+01:23", zone.getID());
assertEquals(DateTimeConstants.MILLIS_PER_HOUR + (23L * DateTimeConstants.MILLIS_PER_MINUTE),
zone.getOffset(TEST_TIME_SUMMER));
zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("GMT-02:00"));
assertEquals("-02:00", zone.getID());
assertEquals((-2L * DateTimeConstants.MILLIS_PER_HOUR), zone.getOffset(TEST_TIME_SUMMER));
zone = DateTimeZone.forTimeZone(TimeZone.getTimeZone("EST"));
assertEquals("America/New_York", zone.getID());
}
public void testTimeZoneConversion() {
TimeZone jdkTimeZone = TimeZone.getTimeZone("GMT-10");
assertEquals("GMT-10:00", jdkTimeZone.getID());
DateTimeZone jodaTimeZone = DateTimeZone.forTimeZone(jdkTimeZone);
assertEquals("-10:00", jodaTimeZone.getID());
assertEquals(jdkTimeZone.getRawOffset(), jodaTimeZone.getOffset(0L));
TimeZone convertedTimeZone = jodaTimeZone.toTimeZone();
assertEquals("GMT-10:00", jdkTimeZone.getID());
assertEquals(jdkTimeZone.getID(), convertedTimeZone.getID());
assertEquals(jdkTimeZone.getRawOffset(), convertedTimeZone.getRawOffset());
}
//-----------------------------------------------------------------------
public void testGetAvailableIDs() {
assertTrue(DateTimeZone.getAvailableIDs().contains("UTC"));
}
//-----------------------------------------------------------------------
public void testProvider() {
try {
assertNotNull(DateTimeZone.getProvider());
Provider provider = DateTimeZone.getProvider();
DateTimeZone.setProvider(null);
assertEquals(provider.getClass(), DateTimeZone.getProvider().getClass());
try {
DateTimeZone.setProvider(new MockNullIDSProvider());
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.setProvider(new MockEmptyIDSProvider());
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.setProvider(new MockNoUTCProvider());
fail();
} catch (IllegalArgumentException ex) {}
try {
DateTimeZone.setProvider(new MockBadUTCProvider());
fail();
} catch (IllegalArgumentException ex) {}
Provider prov = new MockOKProvider();
DateTimeZone.setProvider(prov);
assertSame(prov, DateTimeZone.getProvider());
assertEquals(2, DateTimeZone.getAvailableIDs().size());
assertTrue(DateTimeZone.getAvailableIDs().contains("UTC"));
assertTrue(DateTimeZone.getAvailableIDs().contains("Europe/London"));
} finally {
DateTimeZone.setProvider(null);
assertEquals(ZoneInfoProvider.class, DateTimeZone.getProvider().getClass());
}
try {
System.setProperty("org.joda.time.DateTimeZone.Provider", "org.joda.time.tz.UTCProvider");
DateTimeZone.setProvider(null);
assertEquals(UTCProvider.class, DateTimeZone.getProvider().getClass());
} finally {
System.getProperties().remove("org.joda.time.DateTimeZone.Provider");
DateTimeZone.setProvider(null);
assertEquals(ZoneInfoProvider.class, DateTimeZone.getProvider().getClass());
}
PrintStream syserr = System.err;
try {
System.setProperty("org.joda.time.DateTimeZone.Provider", "xxx");
ByteArrayOutputStream baos = new ByteArrayOutputStream();
System.setErr(new PrintStream(baos));
DateTimeZone.setProvider(null);
assertEquals(ZoneInfoProvider.class, DateTimeZone.getProvider().getClass());
String str = new String(baos.toByteArray());
assertTrue(str.indexOf("java.lang.ClassNotFoundException") >= 0);
} finally {
System.setErr(syserr);
System.getProperties().remove("org.joda.time.DateTimeZone.Provider");
DateTimeZone.setProvider(null);
assertEquals(ZoneInfoProvider.class, DateTimeZone.getProvider().getClass());
}
}
public void testProviderSecurity() {
if (OLD_JDK) {
return;
}
try {
Policy.setPolicy(RESTRICT);
System.setSecurityManager(new SecurityManager());
DateTimeZone.setProvider(new MockOKProvider());
fail();
} catch (SecurityException ex) {
// ok
} finally {
System.setSecurityManager(null);
Policy.setPolicy(ALLOW);
}
}
static class MockNullIDSProvider implements Provider {
public Set getAvailableIDs() {
return null;
}
public DateTimeZone getZone(String id) {
return null;
}
}
static class MockEmptyIDSProvider implements Provider {
public Set getAvailableIDs() {
return new HashSet();
}
public DateTimeZone getZone(String id) {
return null;
}
}
static class MockNoUTCProvider implements Provider {
public Set getAvailableIDs() {
Set set = new HashSet();
set.add("Europe/London");
return set;
}
public DateTimeZone getZone(String id) {
return null;
}
}
static class MockBadUTCProvider implements Provider {
public Set getAvailableIDs() {
Set set = new HashSet();
set.add("UTC");
set.add("Europe/London");
return set;
}
public DateTimeZone getZone(String id) {
return null;
}
}
static class MockOKProvider implements Provider {
public Set getAvailableIDs() {
Set set = new HashSet();
set.add("UTC");
set.add("Europe/London");
return set;
}
public DateTimeZone getZone(String id) {
return DateTimeZone.UTC;
}
}
//-----------------------------------------------------------------------
public void testNameProvider() {
try {
assertNotNull(DateTimeZone.getNameProvider());
NameProvider provider = DateTimeZone.getNameProvider();
DateTimeZone.setNameProvider(null);
assertEquals(provider.getClass(), DateTimeZone.getNameProvider().getClass());
provider = new MockOKButNullNameProvider();
DateTimeZone.setNameProvider(provider);
assertSame(provider, DateTimeZone.getNameProvider());
assertEquals("+00:00", DateTimeZone.UTC.getShortName(TEST_TIME_SUMMER));
assertEquals("+00:00", DateTimeZone.UTC.getName(TEST_TIME_SUMMER));
} finally {
DateTimeZone.setNameProvider(null);
}
try {
System.setProperty("org.joda.time.DateTimeZone.NameProvider", "org.joda.time.tz.DefaultNameProvider");
DateTimeZone.setNameProvider(null);
assertEquals(DefaultNameProvider.class, DateTimeZone.getNameProvider().getClass());
} finally {
System.getProperties().remove("org.joda.time.DateTimeZone.NameProvider");
DateTimeZone.setNameProvider(null);
assertEquals(DefaultNameProvider.class, DateTimeZone.getNameProvider().getClass());
}
PrintStream syserr = System.err;
try {
System.setProperty("org.joda.time.DateTimeZone.NameProvider", "xxx");
ByteArrayOutputStream baos = new ByteArrayOutputStream();
System.setErr(new PrintStream(baos));
DateTimeZone.setNameProvider(null);
assertEquals(DefaultNameProvider.class, DateTimeZone.getNameProvider().getClass());
String str = new String(baos.toByteArray());
assertTrue(str.indexOf("java.lang.ClassNotFoundException") >= 0);
} finally {
System.setErr(syserr);
System.getProperties().remove("org.joda.time.DateTimeZone.NameProvider");
DateTimeZone.setNameProvider(null);
assertEquals(DefaultNameProvider.class, DateTimeZone.getNameProvider().getClass());
}
}
public void testNameProviderSecurity() {
if (OLD_JDK) {
return;
}
try {
Policy.setPolicy(RESTRICT);
System.setSecurityManager(new SecurityManager());
DateTimeZone.setNameProvider(new MockOKButNullNameProvider());
fail();
} catch (SecurityException ex) {
// ok
} finally {
System.setSecurityManager(null);
Policy.setPolicy(ALLOW);
}
}
static class MockOKButNullNameProvider implements NameProvider {
public String getShortName(Locale locale, String id, String nameKey) {
return null;
}
public String getName(Locale locale, String id, String nameKey) {
return null;
}
}
//-----------------------------------------------------------------------
public void testConstructor() {
assertEquals(1, DateTimeZone.class.getDeclaredConstructors().length);
assertTrue(Modifier.isProtected(DateTimeZone.class.getDeclaredConstructors()[0].getModifiers()));
try {
new DateTimeZone(null) {
public String getNameKey(long instant) {
return null;
}
public int getOffset(long instant) {
return 0;
}
public int getStandardOffset(long instant) {
return 0;
}
public boolean isFixed() {
return false;
}
public long nextTransition(long instant) {
return 0;
}
public long previousTransition(long instant) {
return 0;
}
public boolean equals(Object object) {
return false;
}
};
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testGetID() {
DateTimeZone zone = DateTimeZone.forID("Europe/Paris");
assertEquals("Europe/Paris", zone.getID());
}
public void testGetNameKey() {
DateTimeZone zone = DateTimeZone.forID("Europe/London");
assertEquals("BST", zone.getNameKey(TEST_TIME_SUMMER));
assertEquals("GMT", zone.getNameKey(TEST_TIME_WINTER));
}
public void testGetShortName() {}
// Defects4J: flaky method
// public void testGetShortName() {
// DateTimeZone zone = DateTimeZone.forID("Europe/London");
// assertEquals("BST", zone.getShortName(TEST_TIME_SUMMER));
// assertEquals("GMT", zone.getShortName(TEST_TIME_WINTER));
// assertEquals("BST", zone.getShortName(TEST_TIME_SUMMER, Locale.ENGLISH));
// }
public void testGetShortNameProviderName() {
assertEquals(null, DateTimeZone.getNameProvider().getShortName(null, "Europe/London", "BST"));
assertEquals(null, DateTimeZone.getNameProvider().getShortName(Locale.ENGLISH, null, "BST"));
assertEquals(null, DateTimeZone.getNameProvider().getShortName(Locale.ENGLISH, "Europe/London", null));
assertEquals(null, DateTimeZone.getNameProvider().getShortName(null, null, null));
}
public void testGetShortNameNullKey() {
DateTimeZone zone = new MockDateTimeZone("Europe/London");
assertEquals("Europe/London", zone.getShortName(TEST_TIME_SUMMER, Locale.ENGLISH));
}
public void testGetName() {}
// Defects4J: flaky method
// public void testGetName() {
// DateTimeZone zone = DateTimeZone.forID("Europe/London");
// assertEquals("British Summer Time", zone.getName(TEST_TIME_SUMMER));
// assertEquals("Greenwich Mean Time", zone.getName(TEST_TIME_WINTER));
// assertEquals("British Summer Time", zone.getName(TEST_TIME_SUMMER, Locale.ENGLISH));
//
// }
public void testGetNameProviderName() {
assertEquals(null, DateTimeZone.getNameProvider().getName(null, "Europe/London", "BST"));
assertEquals(null, DateTimeZone.getNameProvider().getName(Locale.ENGLISH, null, "BST"));
assertEquals(null, DateTimeZone.getNameProvider().getName(Locale.ENGLISH, "Europe/London", null));
assertEquals(null, DateTimeZone.getNameProvider().getName(null, null, null));
}
public void testGetNameNullKey() {
DateTimeZone zone = new MockDateTimeZone("Europe/London");
assertEquals("Europe/London", zone.getName(TEST_TIME_SUMMER, Locale.ENGLISH));
}
static class MockDateTimeZone extends DateTimeZone {
public MockDateTimeZone(String id) {
super(id);
}
public String getNameKey(long instant) {
return null; // null
}
public int getOffset(long instant) {
return 0;
}
public int getStandardOffset(long instant) {
return 0;
}
public boolean isFixed() {
return false;
}
public long nextTransition(long instant) {
return 0;
}
public long previousTransition(long instant) {
return 0;
}
public boolean equals(Object object) {
return false;
}
}
//-----------------------------------------------------------------------
public void testGetOffset_long() {
DateTimeZone zone = DateTimeZone.forID("Europe/Paris");
assertEquals(2L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffset(TEST_TIME_SUMMER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffset(TEST_TIME_WINTER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getStandardOffset(TEST_TIME_SUMMER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getStandardOffset(TEST_TIME_WINTER));
assertEquals(2L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffsetFromLocal(TEST_TIME_SUMMER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffsetFromLocal(TEST_TIME_WINTER));
assertEquals(false, zone.isStandardOffset(TEST_TIME_SUMMER));
assertEquals(true, zone.isStandardOffset(TEST_TIME_WINTER));
}
public void testGetOffset_RI() {
DateTimeZone zone = DateTimeZone.forID("Europe/Paris");
assertEquals(2L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffset(new Instant(TEST_TIME_SUMMER)));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffset(new Instant(TEST_TIME_WINTER)));
assertEquals(zone.getOffset(DateTimeUtils.currentTimeMillis()), zone.getOffset(null));
}
public void testGetOffsetFixed() {
DateTimeZone zone = DateTimeZone.forID("+01:00");
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffset(TEST_TIME_SUMMER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffset(TEST_TIME_WINTER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getStandardOffset(TEST_TIME_SUMMER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getStandardOffset(TEST_TIME_WINTER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffsetFromLocal(TEST_TIME_SUMMER));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffsetFromLocal(TEST_TIME_WINTER));
assertEquals(true, zone.isStandardOffset(TEST_TIME_SUMMER));
assertEquals(true, zone.isStandardOffset(TEST_TIME_WINTER));
}
public void testGetOffsetFixed_RI() {
DateTimeZone zone = DateTimeZone.forID("+01:00");
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffset(new Instant(TEST_TIME_SUMMER)));
assertEquals(1L * DateTimeConstants.MILLIS_PER_HOUR, zone.getOffset(new Instant(TEST_TIME_WINTER)));
assertEquals(zone.getOffset(DateTimeUtils.currentTimeMillis()), zone.getOffset(null));
}
//-----------------------------------------------------------------------
public void testGetMillisKeepLocal() {
long millisLondon = TEST_TIME_SUMMER;
long millisParis = TEST_TIME_SUMMER - 1L * DateTimeConstants.MILLIS_PER_HOUR;
assertEquals(millisLondon, LONDON.getMillisKeepLocal(LONDON, millisLondon));
assertEquals(millisParis, LONDON.getMillisKeepLocal(LONDON, millisParis));
assertEquals(millisLondon, PARIS.getMillisKeepLocal(PARIS, millisLondon));
assertEquals(millisParis, PARIS.getMillisKeepLocal(PARIS, millisParis));
assertEquals(millisParis, LONDON.getMillisKeepLocal(PARIS, millisLondon));
assertEquals(millisLondon, PARIS.getMillisKeepLocal(LONDON, millisParis));
DateTimeZone zone = DateTimeZone.getDefault();
try {
DateTimeZone.setDefault(LONDON);
assertEquals(millisLondon, PARIS.getMillisKeepLocal(null, millisParis));
} finally {
DateTimeZone.setDefault(zone);
}
}
//-----------------------------------------------------------------------
public void testIsFixed() {
DateTimeZone zone = DateTimeZone.forID("Europe/Paris");
assertEquals(false, zone.isFixed());
assertEquals(true, DateTimeZone.UTC.isFixed());
}
//-----------------------------------------------------------------------
public void testTransitionFixed() {
DateTimeZone zone = DateTimeZone.forID("+01:00");
assertEquals(TEST_TIME_SUMMER, zone.nextTransition(TEST_TIME_SUMMER));
assertEquals(TEST_TIME_WINTER, zone.nextTransition(TEST_TIME_WINTER));
assertEquals(TEST_TIME_SUMMER, zone.previousTransition(TEST_TIME_SUMMER));
assertEquals(TEST_TIME_WINTER, zone.previousTransition(TEST_TIME_WINTER));
}
// //-----------------------------------------------------------------------
// public void testIsLocalDateTimeOverlap_Berlin() {
// DateTimeZone zone = DateTimeZone.forID("Europe/Berlin");
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 10, 28, 1, 0)));
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 10, 28, 1, 59, 59, 99)));
// assertEquals(true, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 10, 28, 2, 0)));
// assertEquals(true, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 10, 28, 2, 30)));
// assertEquals(true, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 10, 28, 2, 59, 59, 99)));
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 10, 28, 3, 0)));
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 10, 28, 4, 0)));
//
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 3, 25, 1, 30))); // before gap
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 3, 25, 2, 30))); // gap
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 3, 25, 3, 30))); // after gap
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 12, 24, 12, 34)));
// }
//
// //-----------------------------------------------------------------------
// public void testIsLocalDateTimeOverlap_NewYork() {
// DateTimeZone zone = DateTimeZone.forID("America/New_York");
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 11, 4, 0, 0)));
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 11, 4, 0, 59, 59, 99)));
// assertEquals(true, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 11, 4, 1, 0)));
// assertEquals(true, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 11, 4, 1, 30)));
// assertEquals(true, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 11, 4, 1, 59, 59, 99)));
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 11, 4, 2, 0)));
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 11, 4, 3, 0)));
//
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 3, 11, 1, 30))); // before gap
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 3, 11, 2, 30))); // gap
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 3, 11, 3, 30))); // after gap
// assertEquals(false, zone.isLocalDateTimeOverlap(new LocalDateTime(2007, 12, 24, 12, 34)));
// }
//-----------------------------------------------------------------------
public void testIsLocalDateTimeGap_Berlin() {
DateTimeZone zone = DateTimeZone.forID("Europe/Berlin");
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 25, 1, 0)));
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 25, 1, 59, 59, 99)));
assertEquals(true, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 25, 2, 0)));
assertEquals(true, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 25, 2, 30)));
assertEquals(true, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 25, 2, 59, 59, 99)));
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 25, 3, 0)));
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 25, 4, 0)));
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 10, 28, 1, 30))); // before overlap
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 10, 28, 2, 30))); // overlap
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 10, 28, 3, 30))); // after overlap
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 12, 24, 12, 34)));
}
//-----------------------------------------------------------------------
public void testIsLocalDateTimeGap_NewYork() {
DateTimeZone zone = DateTimeZone.forID("America/New_York");
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 11, 1, 0)));
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 11, 1, 59, 59, 99)));
assertEquals(true, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 11, 2, 0)));
assertEquals(true, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 11, 2, 30)));
assertEquals(true, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 11, 2, 59, 59, 99)));
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 11, 3, 0)));
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 3, 11, 4, 0)));
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 11, 4, 0, 30))); // before overlap
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 11, 4, 1, 30))); // overlap
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 11, 4, 2, 30))); // after overlap
assertEquals(false, zone.isLocalDateTimeGap(new LocalDateTime(2007, 12, 24, 12, 34)));
}
//-----------------------------------------------------------------------
public void testToTimeZone() {
DateTimeZone zone = DateTimeZone.forID("Europe/Paris");
TimeZone tz = zone.toTimeZone();
assertEquals("Europe/Paris", tz.getID());
}
//-----------------------------------------------------------------------
public void testEqualsHashCode() {
DateTimeZone zone1 = DateTimeZone.forID("Europe/Paris");
DateTimeZone zone2 = DateTimeZone.forID("Europe/Paris");
assertEquals(true, zone1.equals(zone1));
assertEquals(true, zone1.equals(zone2));
assertEquals(true, zone2.equals(zone1));
assertEquals(true, zone2.equals(zone2));
assertEquals(true, zone1.hashCode() == zone2.hashCode());
DateTimeZone zone3 = DateTimeZone.forID("Europe/London");
assertEquals(true, zone3.equals(zone3));
assertEquals(false, zone1.equals(zone3));
assertEquals(false, zone2.equals(zone3));
assertEquals(false, zone3.equals(zone1));
assertEquals(false, zone3.equals(zone2));
assertEquals(false, zone1.hashCode() == zone3.hashCode());
assertEquals(true, zone3.hashCode() == zone3.hashCode());
DateTimeZone zone4 = DateTimeZone.forID("+01:00");
assertEquals(true, zone4.equals(zone4));
assertEquals(false, zone1.equals(zone4));
assertEquals(false, zone2.equals(zone4));
assertEquals(false, zone3.equals(zone4));
assertEquals(false, zone4.equals(zone1));
assertEquals(false, zone4.equals(zone2));
assertEquals(false, zone4.equals(zone3));
assertEquals(false, zone1.hashCode() == zone4.hashCode());
assertEquals(true, zone4.hashCode() == zone4.hashCode());
DateTimeZone zone5 = DateTimeZone.forID("+02:00");
assertEquals(true, zone5.equals(zone5));
assertEquals(false, zone1.equals(zone5));
assertEquals(false, zone2.equals(zone5));
assertEquals(false, zone3.equals(zone5));
assertEquals(false, zone4.equals(zone5));
assertEquals(false, zone5.equals(zone1));
assertEquals(false, zone5.equals(zone2));
assertEquals(false, zone5.equals(zone3));
assertEquals(false, zone5.equals(zone4));
assertEquals(false, zone1.hashCode() == zone5.hashCode());
assertEquals(true, zone5.hashCode() == zone5.hashCode());
}
//-----------------------------------------------------------------------
public void testToString() {
DateTimeZone zone = DateTimeZone.forID("Europe/Paris");
assertEquals("Europe/Paris", zone.toString());
assertEquals("UTC", DateTimeZone.UTC.toString());
}
//-----------------------------------------------------------------------
public void testSerialization1() throws Exception {
DateTimeZone zone = DateTimeZone.forID("Europe/Paris");
ByteArrayOutputStream baos = new ByteArrayOutputStream();
ObjectOutputStream oos = new ObjectOutputStream(baos);
oos.writeObject(zone);
byte[] bytes = baos.toByteArray();
oos.close();
ByteArrayInputStream bais = new ByteArrayInputStream(bytes);
ObjectInputStream ois = new ObjectInputStream(bais);
DateTimeZone result = (DateTimeZone) ois.readObject();
ois.close();
assertSame(zone, result);
}
//-----------------------------------------------------------------------
public void testSerialization2() throws Exception {
DateTimeZone zone = DateTimeZone.forID("+01:00");
ByteArrayOutputStream baos = new ByteArrayOutputStream();
ObjectOutputStream oos = new ObjectOutputStream(baos);
oos.writeObject(zone);
byte[] bytes = baos.toByteArray();
oos.close();
ByteArrayInputStream bais = new ByteArrayInputStream(bytes);
ObjectInputStream ois = new ObjectInputStream(bais);
DateTimeZone result = (DateTimeZone) ois.readObject();
ois.close();
assertSame(zone, result);
}
public void testCommentParse() throws Exception {
// A bug in ZoneInfoCompiler's handling of comments broke Europe/Athens
// after 1980. This test is included to make sure it doesn't break again.
DateTimeZone zone = DateTimeZone.forID("Europe/Athens");
DateTime dt = new DateTime(2005, 5, 5, 20, 10, 15, 0, zone);
assertEquals(1115313015000L, dt.getMillis());
}
public void testPatchedNameKeysLondon() throws Exception {
// the tz database does not have unique name keys [1716305]
DateTimeZone zone = DateTimeZone.forID("Europe/London");
DateTime now = new DateTime(2007, 1, 1, 0, 0, 0, 0);
String str1 = zone.getName(now.getMillis());
String str2 = zone.getName(now.plusMonths(6).getMillis());
assertEquals(false, str1.equals(str2));
}
public void testPatchedNameKeysSydney() throws Exception {
// the tz database does not have unique name keys [1716305]
DateTimeZone zone = DateTimeZone.forID("Australia/Sydney");
DateTime now = new DateTime(2007, 1, 1, 0, 0, 0, 0);
String str1 = zone.getName(now.getMillis());
String str2 = zone.getName(now.plusMonths(6).getMillis());
assertEquals(false, str1.equals(str2));
}
public void testPatchedNameKeysSydneyHistoric() throws Exception {
// the tz database does not have unique name keys [1716305]
DateTimeZone zone = DateTimeZone.forID("Australia/Sydney");
DateTime now = new DateTime(1996, 1, 1, 0, 0, 0, 0);
String str1 = zone.getName(now.getMillis());
String str2 = zone.getName(now.plusMonths(6).getMillis());
assertEquals(false, str1.equals(str2));
}
public void testPatchedNameKeysGazaHistoric() throws Exception {
// the tz database does not have unique name keys [1716305]
DateTimeZone zone = DateTimeZone.forID("Africa/Johannesburg");
DateTime now = new DateTime(1943, 1, 1, 0, 0, 0, 0);
String str1 = zone.getName(now.getMillis());
String str2 = zone.getName(now.plusMonths(6).getMillis());
assertEquals(false, str1.equals(str2));
}
} |
||
public void testCompressedHeaderWithNonDefaultDictionarySize() throws Exception {
SevenZFile sevenZFile = new SevenZFile(getFile("COMPRESS-256.7z"));
try {
int count = 0;
while (sevenZFile.getNextEntry() != null) {
count++;
}
assertEquals(446, count);
} finally {
sevenZFile.close();
}
} | org.apache.commons.compress.archivers.sevenz.SevenZFileTest::testCompressedHeaderWithNonDefaultDictionarySize | src/test/java/org/apache/commons/compress/archivers/sevenz/SevenZFileTest.java | 88 | src/test/java/org/apache/commons/compress/archivers/sevenz/SevenZFileTest.java | testCompressedHeaderWithNonDefaultDictionarySize | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package org.apache.commons.compress.archivers.sevenz;
import java.io.File;
import java.io.IOException;
import java.security.NoSuchAlgorithmException;
import javax.crypto.Cipher;
import org.apache.commons.compress.AbstractTestCase;
public class SevenZFileTest extends AbstractTestCase {
private static final String TEST2_CONTENT = "<?xml version = '1.0'?>\r\n<!DOCTYPE"
+ " connections>\r\n<meinxml>\r\n\t<leer />\r\n</meinxml>\n";
public void testAllEmptyFilesArchive() throws Exception {
SevenZFile archive = new SevenZFile(getFile("7z-empty-mhc-off.7z"));
try {
assertNotNull(archive.getNextEntry());
} finally {
archive.close();
}
}
public void testHelloWorldHeaderCompressionOffCopy() throws Exception {
checkHelloWorld("7z-hello-mhc-off-copy.7z");
}
public void testHelloWorldHeaderCompressionOffLZMA2() throws Exception {
checkHelloWorld("7z-hello-mhc-off-lzma2.7z");
}
public void test7zUnarchive() throws Exception {
test7zUnarchive(getFile("bla.7z"));
}
public void test7zDeflateUnarchive() throws Exception {
test7zUnarchive(getFile("bla.deflate.7z"));
}
public void test7zDecryptUnarchive() throws Exception {
if (isStrongCryptoAvailable()) {
test7zUnarchive(getFile("bla.encrypted.7z"), "foo".getBytes("UTF-16LE"));
}
}
private void test7zUnarchive(File f) throws Exception {
test7zUnarchive(f, null);
}
public void testEncryptedArchiveRequiresPassword() throws Exception {
try {
new SevenZFile(getFile("bla.encrypted.7z"));
fail("shouldn't decrypt without a password");
} catch (IOException ex) {
assertEquals("Cannot read encrypted files without a password",
ex.getMessage());
}
}
/**
* @see "https://issues.apache.org/jira/browse/COMPRESS-256"
*/
public void testCompressedHeaderWithNonDefaultDictionarySize() throws Exception {
SevenZFile sevenZFile = new SevenZFile(getFile("COMPRESS-256.7z"));
try {
int count = 0;
while (sevenZFile.getNextEntry() != null) {
count++;
}
assertEquals(446, count);
} finally {
sevenZFile.close();
}
}
private void test7zUnarchive(File f, byte[] password) throws Exception {
SevenZFile sevenZFile = new SevenZFile(f, password);
try {
SevenZArchiveEntry entry = sevenZFile.getNextEntry();
assertEquals("test1.xml", entry.getName());
entry = sevenZFile.getNextEntry();
assertEquals("test2.xml", entry.getName());
byte[] contents = new byte[(int)entry.getSize()];
int off = 0;
while ((off < contents.length)) {
int bytesRead = sevenZFile.read(contents, off, contents.length - off);
assert(bytesRead >= 0);
off += bytesRead;
}
assertEquals(TEST2_CONTENT, new String(contents, "UTF-8"));
assertNull(sevenZFile.getNextEntry());
} finally {
sevenZFile.close();
}
}
private void checkHelloWorld(final String filename) throws Exception {
SevenZFile sevenZFile = new SevenZFile(getFile(filename));
try {
SevenZArchiveEntry entry = sevenZFile.getNextEntry();
assertEquals("Hello world.txt", entry.getName());
byte[] contents = new byte[(int)entry.getSize()];
int off = 0;
while ((off < contents.length)) {
int bytesRead = sevenZFile.read(contents, off, contents.length - off);
assert(bytesRead >= 0);
off += bytesRead;
}
assertEquals("Hello, world!\n", new String(contents, "UTF-8"));
assertNull(sevenZFile.getNextEntry());
} finally {
sevenZFile.close();
}
}
private static boolean isStrongCryptoAvailable() throws NoSuchAlgorithmException {
return Cipher.getMaxAllowedKeyLength("AES/ECB/PKCS5Padding") >= 256;
}
} | // You are a professional Java test case writer, please create a test case named `testCompressedHeaderWithNonDefaultDictionarySize` for the issue `Compress-COMPRESS-256`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Compress-COMPRESS-256
//
// ## Issue-Title:
// 7z: 16 MB dictionary is too big
//
// ## Issue-Description:
//
// I created an archiv with 7zip 9.20 containing the compress-1.7-src directory. Also tried it with 1.6 version and directory. I
//
//
// downloaded the zip file and reziped it as 7z. The standard setting where used:
//
// Compression level: normal
//
// Compression method: lzma2
//
// Dictionary size: 16 MB
//
// Word size: 32
//
// Solid Block size: 2 GB
//
//
// I get an exception if I try to open the file with the simple line of code:
//
// SevenZFile input = new SevenZFile(new File(arcName));
//
//
// Maybe it is a bug in the tukaani library, but I do not know how to report it to them.
//
// The exception thrown:
//
//
// org.tukaani.xz.UnsupportedOptionsException: LZMA dictionary is too big for this implementation
//
// at org.tukaani.xz.LZMAInputStream.initialize(Unknown Source)
//
// at org.tukaani.xz.LZMAInputStream.<init>(Unknown Source)
//
// at org.apache.commons.compress.archivers.sevenz.Coders$LZMADecoder.decode(Coders.java:117)
//
// at org.apache.commons.compress.archivers.sevenz.Coders.addDecoder(Coders.java:48)
//
// at org.apache.commons.compress.archivers.sevenz.SevenZFile.readEncodedHeader(SevenZFile.java:278)
//
// at org.apache.commons.compress.archivers.sevenz.SevenZFile.readHeaders(SevenZFile.java:190)
//
// at org.apache.commons.compress.archivers.sevenz.SevenZFile.<init>(SevenZFile.java:94)
//
// at org.apache.commons.compress.archivers.sevenz.SevenZFile.<init>(SevenZFile.java:116)
//
// at compress.SevenZipError.main(SevenZipError.java:28)
//
//
//
//
//
public void testCompressedHeaderWithNonDefaultDictionarySize() throws Exception {
| 88 | /**
* @see "https://issues.apache.org/jira/browse/COMPRESS-256"
*/ | 23 | 77 | src/test/java/org/apache/commons/compress/archivers/sevenz/SevenZFileTest.java | src/test/java | ```markdown
## Issue-ID: Compress-COMPRESS-256
## Issue-Title:
7z: 16 MB dictionary is too big
## Issue-Description:
I created an archiv with 7zip 9.20 containing the compress-1.7-src directory. Also tried it with 1.6 version and directory. I
downloaded the zip file and reziped it as 7z. The standard setting where used:
Compression level: normal
Compression method: lzma2
Dictionary size: 16 MB
Word size: 32
Solid Block size: 2 GB
I get an exception if I try to open the file with the simple line of code:
SevenZFile input = new SevenZFile(new File(arcName));
Maybe it is a bug in the tukaani library, but I do not know how to report it to them.
The exception thrown:
org.tukaani.xz.UnsupportedOptionsException: LZMA dictionary is too big for this implementation
at org.tukaani.xz.LZMAInputStream.initialize(Unknown Source)
at org.tukaani.xz.LZMAInputStream.<init>(Unknown Source)
at org.apache.commons.compress.archivers.sevenz.Coders$LZMADecoder.decode(Coders.java:117)
at org.apache.commons.compress.archivers.sevenz.Coders.addDecoder(Coders.java:48)
at org.apache.commons.compress.archivers.sevenz.SevenZFile.readEncodedHeader(SevenZFile.java:278)
at org.apache.commons.compress.archivers.sevenz.SevenZFile.readHeaders(SevenZFile.java:190)
at org.apache.commons.compress.archivers.sevenz.SevenZFile.<init>(SevenZFile.java:94)
at org.apache.commons.compress.archivers.sevenz.SevenZFile.<init>(SevenZFile.java:116)
at compress.SevenZipError.main(SevenZipError.java:28)
```
You are a professional Java test case writer, please create a test case named `testCompressedHeaderWithNonDefaultDictionarySize` for the issue `Compress-COMPRESS-256`, utilizing the provided issue report information and the following function signature.
```java
public void testCompressedHeaderWithNonDefaultDictionarySize() throws Exception {
```
| 77 | [
"org.apache.commons.compress.archivers.sevenz.Coders"
] | 81c80475325e99fc609ea6210d6ce8287a834409b124cebb305936f4b8becdbb | public void testCompressedHeaderWithNonDefaultDictionarySize() throws Exception | // You are a professional Java test case writer, please create a test case named `testCompressedHeaderWithNonDefaultDictionarySize` for the issue `Compress-COMPRESS-256`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Compress-COMPRESS-256
//
// ## Issue-Title:
// 7z: 16 MB dictionary is too big
//
// ## Issue-Description:
//
// I created an archiv with 7zip 9.20 containing the compress-1.7-src directory. Also tried it with 1.6 version and directory. I
//
//
// downloaded the zip file and reziped it as 7z. The standard setting where used:
//
// Compression level: normal
//
// Compression method: lzma2
//
// Dictionary size: 16 MB
//
// Word size: 32
//
// Solid Block size: 2 GB
//
//
// I get an exception if I try to open the file with the simple line of code:
//
// SevenZFile input = new SevenZFile(new File(arcName));
//
//
// Maybe it is a bug in the tukaani library, but I do not know how to report it to them.
//
// The exception thrown:
//
//
// org.tukaani.xz.UnsupportedOptionsException: LZMA dictionary is too big for this implementation
//
// at org.tukaani.xz.LZMAInputStream.initialize(Unknown Source)
//
// at org.tukaani.xz.LZMAInputStream.<init>(Unknown Source)
//
// at org.apache.commons.compress.archivers.sevenz.Coders$LZMADecoder.decode(Coders.java:117)
//
// at org.apache.commons.compress.archivers.sevenz.Coders.addDecoder(Coders.java:48)
//
// at org.apache.commons.compress.archivers.sevenz.SevenZFile.readEncodedHeader(SevenZFile.java:278)
//
// at org.apache.commons.compress.archivers.sevenz.SevenZFile.readHeaders(SevenZFile.java:190)
//
// at org.apache.commons.compress.archivers.sevenz.SevenZFile.<init>(SevenZFile.java:94)
//
// at org.apache.commons.compress.archivers.sevenz.SevenZFile.<init>(SevenZFile.java:116)
//
// at compress.SevenZipError.main(SevenZipError.java:28)
//
//
//
//
//
| Compress | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package org.apache.commons.compress.archivers.sevenz;
import java.io.File;
import java.io.IOException;
import java.security.NoSuchAlgorithmException;
import javax.crypto.Cipher;
import org.apache.commons.compress.AbstractTestCase;
public class SevenZFileTest extends AbstractTestCase {
private static final String TEST2_CONTENT = "<?xml version = '1.0'?>\r\n<!DOCTYPE"
+ " connections>\r\n<meinxml>\r\n\t<leer />\r\n</meinxml>\n";
public void testAllEmptyFilesArchive() throws Exception {
SevenZFile archive = new SevenZFile(getFile("7z-empty-mhc-off.7z"));
try {
assertNotNull(archive.getNextEntry());
} finally {
archive.close();
}
}
public void testHelloWorldHeaderCompressionOffCopy() throws Exception {
checkHelloWorld("7z-hello-mhc-off-copy.7z");
}
public void testHelloWorldHeaderCompressionOffLZMA2() throws Exception {
checkHelloWorld("7z-hello-mhc-off-lzma2.7z");
}
public void test7zUnarchive() throws Exception {
test7zUnarchive(getFile("bla.7z"));
}
public void test7zDeflateUnarchive() throws Exception {
test7zUnarchive(getFile("bla.deflate.7z"));
}
public void test7zDecryptUnarchive() throws Exception {
if (isStrongCryptoAvailable()) {
test7zUnarchive(getFile("bla.encrypted.7z"), "foo".getBytes("UTF-16LE"));
}
}
private void test7zUnarchive(File f) throws Exception {
test7zUnarchive(f, null);
}
public void testEncryptedArchiveRequiresPassword() throws Exception {
try {
new SevenZFile(getFile("bla.encrypted.7z"));
fail("shouldn't decrypt without a password");
} catch (IOException ex) {
assertEquals("Cannot read encrypted files without a password",
ex.getMessage());
}
}
/**
* @see "https://issues.apache.org/jira/browse/COMPRESS-256"
*/
public void testCompressedHeaderWithNonDefaultDictionarySize() throws Exception {
SevenZFile sevenZFile = new SevenZFile(getFile("COMPRESS-256.7z"));
try {
int count = 0;
while (sevenZFile.getNextEntry() != null) {
count++;
}
assertEquals(446, count);
} finally {
sevenZFile.close();
}
}
private void test7zUnarchive(File f, byte[] password) throws Exception {
SevenZFile sevenZFile = new SevenZFile(f, password);
try {
SevenZArchiveEntry entry = sevenZFile.getNextEntry();
assertEquals("test1.xml", entry.getName());
entry = sevenZFile.getNextEntry();
assertEquals("test2.xml", entry.getName());
byte[] contents = new byte[(int)entry.getSize()];
int off = 0;
while ((off < contents.length)) {
int bytesRead = sevenZFile.read(contents, off, contents.length - off);
assert(bytesRead >= 0);
off += bytesRead;
}
assertEquals(TEST2_CONTENT, new String(contents, "UTF-8"));
assertNull(sevenZFile.getNextEntry());
} finally {
sevenZFile.close();
}
}
private void checkHelloWorld(final String filename) throws Exception {
SevenZFile sevenZFile = new SevenZFile(getFile(filename));
try {
SevenZArchiveEntry entry = sevenZFile.getNextEntry();
assertEquals("Hello world.txt", entry.getName());
byte[] contents = new byte[(int)entry.getSize()];
int off = 0;
while ((off < contents.length)) {
int bytesRead = sevenZFile.read(contents, off, contents.length - off);
assert(bytesRead >= 0);
off += bytesRead;
}
assertEquals("Hello, world!\n", new String(contents, "UTF-8"));
assertNull(sevenZFile.getNextEntry());
} finally {
sevenZFile.close();
}
}
private static boolean isStrongCryptoAvailable() throws NoSuchAlgorithmException {
return Cipher.getMaxAllowedKeyLength("AES/ECB/PKCS5Padding") >= 256;
}
} |
|
public void testDependencySortingWhitespaceMode() {
args.add("--manage_closure_dependencies");
args.add("--compilation_level=WHITESPACE_ONLY");
test(new String[] {
"goog.require('beer');",
"goog.provide('beer');\ngoog.require('hops');",
"goog.provide('hops');",
},
new String[] {
"goog.provide('hops');",
"goog.provide('beer');\ngoog.require('hops');",
"goog.require('beer');"
});
} | com.google.javascript.jscomp.CommandLineRunnerTest::testDependencySortingWhitespaceMode | test/com/google/javascript/jscomp/CommandLineRunnerTest.java | 636 | test/com/google/javascript/jscomp/CommandLineRunnerTest.java | testDependencySortingWhitespaceMode | /*
* Copyright 2009 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import com.google.common.base.Function;
import com.google.common.base.Joiner;
import com.google.common.base.Preconditions;
import com.google.common.base.Supplier;
import com.google.common.base.Suppliers;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.Lists;
import com.google.common.collect.Maps;
import com.google.javascript.jscomp.AbstractCommandLineRunner.FlagUsageException;
import com.google.javascript.jscomp.CompilerOptions.LanguageMode;
import com.google.javascript.rhino.Node;
import junit.framework.TestCase;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.PrintStream;
import java.util.List;
import java.util.Map;
/**
* Tests for {@link CommandLineRunner}.
*
* @author [email protected] (Nick Santos)
*/
public class CommandLineRunnerTest extends TestCase {
private Compiler lastCompiler = null;
private CommandLineRunner lastCommandLineRunner = null;
private List<Integer> exitCodes = null;
private ByteArrayOutputStream outReader = null;
private ByteArrayOutputStream errReader = null;
private Map<Integer,String> filenames;
// If set, this will be appended to the end of the args list.
// For testing args parsing.
private String lastArg = null;
// If set to true, uses comparison by string instead of by AST.
private boolean useStringComparison = false;
private ModulePattern useModules = ModulePattern.NONE;
private enum ModulePattern {
NONE,
CHAIN,
STAR
}
private List<String> args = Lists.newArrayList();
/** Externs for the test */
private final List<SourceFile> DEFAULT_EXTERNS = ImmutableList.of(
SourceFile.fromCode("externs",
"var arguments;"
+ "/**\n"
+ " * @constructor\n"
+ " * @param {...*} var_args\n"
+ " */\n"
+ "function Function(var_args) {}\n"
+ "/**\n"
+ " * @param {...*} var_args\n"
+ " * @return {*}\n"
+ " */\n"
+ "Function.prototype.call = function(var_args) {};"
+ "/**\n"
+ " * @constructor\n"
+ " * @param {...*} var_args\n"
+ " * @return {!Array}\n"
+ " */\n"
+ "function Array(var_args) {}"
+ "/**\n"
+ " * @param {*=} opt_begin\n"
+ " * @param {*=} opt_end\n"
+ " * @return {!Array}\n"
+ " * @this {Object}\n"
+ " */\n"
+ "Array.prototype.slice = function(opt_begin, opt_end) {};"
+ "/** @constructor */ function Window() {}\n"
+ "/** @type {string} */ Window.prototype.name;\n"
+ "/** @type {Window} */ var window;"
+ "/** @constructor */ function Element() {}"
+ "Element.prototype.offsetWidth;"
+ "/** @nosideeffects */ function noSideEffects() {}")
);
private List<SourceFile> externs;
@Override
public void setUp() throws Exception {
super.setUp();
externs = DEFAULT_EXTERNS;
filenames = Maps.newHashMap();
lastCompiler = null;
lastArg = null;
outReader = new ByteArrayOutputStream();
errReader = new ByteArrayOutputStream();
useStringComparison = false;
useModules = ModulePattern.NONE;
args.clear();
exitCodes = Lists.newArrayList();
}
@Override
public void tearDown() throws Exception {
super.tearDown();
}
public void testWarningGuardOrdering1() {
args.add("--jscomp_error=globalThis");
args.add("--jscomp_off=globalThis");
testSame("function f() { this.a = 3; }");
}
public void testWarningGuardOrdering2() {
args.add("--jscomp_off=globalThis");
args.add("--jscomp_error=globalThis");
test("function f() { this.a = 3; }", CheckGlobalThis.GLOBAL_THIS);
}
public void testWarningGuardOrdering3() {
args.add("--jscomp_warning=globalThis");
args.add("--jscomp_off=globalThis");
testSame("function f() { this.a = 3; }");
}
public void testWarningGuardOrdering4() {
args.add("--jscomp_off=globalThis");
args.add("--jscomp_warning=globalThis");
test("function f() { this.a = 3; }", CheckGlobalThis.GLOBAL_THIS);
}
public void testCheckGlobalThisOffByDefault() {
testSame("function f() { this.a = 3; }");
}
public void testCheckGlobalThisOnWithAdvancedMode() {
args.add("--compilation_level=ADVANCED_OPTIMIZATIONS");
test("function f() { this.a = 3; }", CheckGlobalThis.GLOBAL_THIS);
}
public void testCheckGlobalThisOnWithErrorFlag() {
args.add("--jscomp_error=globalThis");
test("function f() { this.a = 3; }", CheckGlobalThis.GLOBAL_THIS);
}
public void testCheckGlobalThisOff() {
args.add("--warning_level=VERBOSE");
args.add("--jscomp_off=globalThis");
testSame("function f() { this.a = 3; }");
}
public void testTypeCheckingOffByDefault() {
test("function f(x) { return x; } f();",
"function f(a) { return a; } f();");
}
public void testReflectedMethods() {
args.add("--compilation_level=ADVANCED_OPTIMIZATIONS");
test(
"/** @constructor */" +
"function Foo() {}" +
"Foo.prototype.handle = function(x, y) { alert(y); };" +
"var x = goog.reflect.object(Foo, {handle: 1});" +
"for (var i in x) { x[i].call(x); }" +
"window['Foo'] = Foo;",
"function a() {}" +
"a.prototype.a = function(e, d) { alert(d); };" +
"var b = goog.c.b(a, {a: 1}),c;" +
"for (c in b) { b[c].call(b); }" +
"window.Foo = a;");
}
public void testTypeCheckingOnWithVerbose() {
args.add("--warning_level=VERBOSE");
test("function f(x) { return x; } f();", TypeCheck.WRONG_ARGUMENT_COUNT);
}
public void testTypeParsingOffByDefault() {
testSame("/** @return {number */ function f(a) { return a; }");
}
public void testTypeParsingOnWithVerbose() {
args.add("--warning_level=VERBOSE");
test("/** @return {number */ function f(a) { return a; }",
RhinoErrorReporter.TYPE_PARSE_ERROR);
test("/** @return {n} */ function f(a) { return a; }",
RhinoErrorReporter.TYPE_PARSE_ERROR);
}
public void testTypeCheckOverride1() {
args.add("--warning_level=VERBOSE");
args.add("--jscomp_off=checkTypes");
testSame("var x = x || {}; x.f = function() {}; x.f(3);");
}
public void testTypeCheckOverride2() {
args.add("--warning_level=DEFAULT");
testSame("var x = x || {}; x.f = function() {}; x.f(3);");
args.add("--jscomp_warning=checkTypes");
test("var x = x || {}; x.f = function() {}; x.f(3);",
TypeCheck.WRONG_ARGUMENT_COUNT);
}
public void testCheckSymbolsOffForDefault() {
args.add("--warning_level=DEFAULT");
test("x = 3; var y; var y;", "x=3; var y;");
}
public void testCheckSymbolsOnForVerbose() {
args.add("--warning_level=VERBOSE");
test("x = 3;", VarCheck.UNDEFINED_VAR_ERROR);
test("var y; var y;", SyntacticScopeCreator.VAR_MULTIPLY_DECLARED_ERROR);
}
public void testCheckSymbolsOverrideForVerbose() {
args.add("--warning_level=VERBOSE");
args.add("--jscomp_off=undefinedVars");
testSame("x = 3;");
}
public void testCheckSymbolsOverrideForQuiet() {
args.add("--warning_level=QUIET");
args.add("--jscomp_error=undefinedVars");
test("x = 3;", VarCheck.UNDEFINED_VAR_ERROR);
}
public void testCheckUndefinedProperties1() {
args.add("--warning_level=VERBOSE");
args.add("--jscomp_error=missingProperties");
test("var x = {}; var y = x.bar;", TypeCheck.INEXISTENT_PROPERTY);
}
public void testCheckUndefinedProperties2() {
args.add("--warning_level=VERBOSE");
args.add("--jscomp_off=missingProperties");
test("var x = {}; var y = x.bar;", CheckGlobalNames.UNDEFINED_NAME_WARNING);
}
public void testCheckUndefinedProperties3() {
args.add("--warning_level=VERBOSE");
test("function f() {var x = {}; var y = x.bar;}",
TypeCheck.INEXISTENT_PROPERTY);
}
public void testDuplicateParams() {
test("function f(a, a) {}", RhinoErrorReporter.DUPLICATE_PARAM);
assertTrue(lastCompiler.hasHaltingErrors());
}
public void testDefineFlag() {
args.add("--define=FOO");
args.add("--define=\"BAR=5\"");
args.add("--D"); args.add("CCC");
args.add("-D"); args.add("DDD");
test("/** @define {boolean} */ var FOO = false;" +
"/** @define {number} */ var BAR = 3;" +
"/** @define {boolean} */ var CCC = false;" +
"/** @define {boolean} */ var DDD = false;",
"var FOO = !0, BAR = 5, CCC = !0, DDD = !0;");
}
public void testDefineFlag2() {
args.add("--define=FOO='x\"'");
test("/** @define {string} */ var FOO = \"a\";",
"var FOO = \"x\\\"\";");
}
public void testDefineFlag3() {
args.add("--define=FOO=\"x'\"");
test("/** @define {string} */ var FOO = \"a\";",
"var FOO = \"x'\";");
}
public void testScriptStrictModeNoWarning() {
test("'use strict';", "");
test("'no use strict';", CheckSideEffects.USELESS_CODE_ERROR);
}
public void testFunctionStrictModeNoWarning() {
test("function f() {'use strict';}", "function f() {}");
test("function f() {'no use strict';}",
CheckSideEffects.USELESS_CODE_ERROR);
}
public void testQuietMode() {
args.add("--warning_level=DEFAULT");
test("/** @const \n * @const */ var x;",
RhinoErrorReporter.PARSE_ERROR);
args.add("--warning_level=QUIET");
testSame("/** @const \n * @const */ var x;");
}
public void testProcessClosurePrimitives() {
test("var goog = {}; goog.provide('goog.dom');",
"var goog = {dom:{}};");
args.add("--process_closure_primitives=false");
testSame("var goog = {}; goog.provide('goog.dom');");
}
public void testGetMsgWiring() throws Exception {
test("var goog = {}; goog.getMsg = function(x) { return x; };" +
"/** @desc A real foo. */ var MSG_FOO = goog.getMsg('foo');",
"var goog={getMsg:function(a){return a}}, " +
"MSG_FOO=goog.getMsg('foo');");
args.add("--compilation_level=ADVANCED_OPTIMIZATIONS");
test("var goog = {}; goog.getMsg = function(x) { return x; };" +
"/** @desc A real foo. */ var MSG_FOO = goog.getMsg('foo');" +
"window['foo'] = MSG_FOO;",
"window.foo = 'foo';");
}
public void testCssNameWiring() throws Exception {
test("var goog = {}; goog.getCssName = function() {};" +
"goog.setCssNameMapping = function() {};" +
"goog.setCssNameMapping({'goog': 'a', 'button': 'b'});" +
"var a = goog.getCssName('goog-button');" +
"var b = goog.getCssName('css-button');" +
"var c = goog.getCssName('goog-menu');" +
"var d = goog.getCssName('css-menu');",
"var goog = { getCssName: function() {}," +
" setCssNameMapping: function() {} }," +
" a = 'a-b'," +
" b = 'css-b'," +
" c = 'a-menu'," +
" d = 'css-menu';");
}
//////////////////////////////////////////////////////////////////////////////
// Integration tests
public void testIssue70a() {
test("function foo({}) {}", RhinoErrorReporter.PARSE_ERROR);
}
public void testIssue70b() {
test("function foo([]) {}", RhinoErrorReporter.PARSE_ERROR);
}
public void testIssue81() {
args.add("--compilation_level=ADVANCED_OPTIMIZATIONS");
useStringComparison = true;
test("eval('1'); var x = eval; x('2');",
"eval(\"1\");(0,eval)(\"2\");");
}
public void testIssue115() {
args.add("--compilation_level=SIMPLE_OPTIMIZATIONS");
args.add("--jscomp_off=es5Strict");
args.add("--warning_level=VERBOSE");
test("function f() { " +
" var arguments = Array.prototype.slice.call(arguments, 0);" +
" return arguments[0]; " +
"}",
"function f() { " +
" arguments = Array.prototype.slice.call(arguments, 0);" +
" return arguments[0]; " +
"}");
}
public void testIssue297() {
args.add("--compilation_level=SIMPLE_OPTIMIZATIONS");
test("function f(p) {" +
" var x;" +
" return ((x=p.id) && (x=parseInt(x.substr(1))) && x>0);" +
"}",
"function f(b) {" +
" var a;" +
" return ((a=b.id) && (a=parseInt(a.substr(1))) && 0<a);" +
"}");
}
public void testHiddenSideEffect() {
args.add("--compilation_level=ADVANCED_OPTIMIZATIONS");
test("element.offsetWidth;",
"element.offsetWidth", CheckSideEffects.USELESS_CODE_ERROR);
}
public void testIssue504() {
args.add("--compilation_level=ADVANCED_OPTIMIZATIONS");
test("void function() { alert('hi'); }();",
"alert('hi');void 0", CheckSideEffects.USELESS_CODE_ERROR);
}
public void testIssue601() {
args.add("--compilation_level=WHITESPACE_ONLY");
test("function f() { return '\\v' == 'v'; } window['f'] = f;",
"function f(){return'\\v'=='v'}window['f']=f");
}
public void testIssue601b() {
args.add("--compilation_level=ADVANCED_OPTIMIZATIONS");
test("function f() { return '\\v' == 'v'; } window['f'] = f;",
"window.f=function(){return'\\v'=='v'}");
}
public void testIssue601c() {
args.add("--compilation_level=ADVANCED_OPTIMIZATIONS");
test("function f() { return '\\u000B' == 'v'; } window['f'] = f;",
"window.f=function(){return'\\u000B'=='v'}");
}
public void testDebugFlag1() {
args.add("--compilation_level=SIMPLE_OPTIMIZATIONS");
args.add("--debug=false");
test("function foo(a) {}",
"function foo() {}");
}
public void testDebugFlag2() {
args.add("--compilation_level=SIMPLE_OPTIMIZATIONS");
args.add("--debug=true");
test("function foo(a) {alert(a)}",
"function foo($a$$) {alert($a$$)}");
}
public void testDebugFlag3() {
args.add("--compilation_level=ADVANCED_OPTIMIZATIONS");
args.add("--warning_level=QUIET");
args.add("--debug=false");
test("function Foo() {}" +
"Foo.x = 1;" +
"function f() {throw new Foo().x;} f();",
"throw (new function() {}).a;");
}
public void testDebugFlag4() {
args.add("--compilation_level=ADVANCED_OPTIMIZATIONS");
args.add("--warning_level=QUIET");
args.add("--debug=true");
test("function Foo() {}" +
"Foo.x = 1;" +
"function f() {throw new Foo().x;} f();",
"throw (new function Foo() {}).$x$;");
}
public void testBooleanFlag1() {
args.add("--compilation_level=SIMPLE_OPTIMIZATIONS");
args.add("--debug");
test("function foo(a) {alert(a)}",
"function foo($a$$) {alert($a$$)}");
}
public void testBooleanFlag2() {
args.add("--debug");
args.add("--compilation_level=SIMPLE_OPTIMIZATIONS");
test("function foo(a) {alert(a)}",
"function foo($a$$) {alert($a$$)}");
}
public void testHelpFlag() {
args.add("--help");
assertFalse(
createCommandLineRunner(
new String[] {"function f() {}"}).shouldRunCompiler());
}
public void testExternsLifting1() throws Exception{
String code = "/** @externs */ function f() {}";
test(new String[] {code},
new String[] {});
assertEquals(2, lastCompiler.getExternsForTesting().size());
CompilerInput extern = lastCompiler.getExternsForTesting().get(1);
assertNull(extern.getModule());
assertTrue(extern.isExtern());
assertEquals(code, extern.getCode());
assertEquals(1, lastCompiler.getInputsForTesting().size());
CompilerInput input = lastCompiler.getInputsForTesting().get(0);
assertNotNull(input.getModule());
assertFalse(input.isExtern());
assertEquals("", input.getCode());
}
public void testExternsLifting2() {
args.add("--warning_level=VERBOSE");
test(new String[] {"/** @externs */ function f() {}", "f(3);"},
new String[] {"f(3);"},
TypeCheck.WRONG_ARGUMENT_COUNT);
}
public void testSourceSortingOff() {
args.add("--compilation_level=WHITESPACE_ONLY");
testSame(
new String[] {
"goog.require('beer');",
"goog.provide('beer');"
});
}
public void testSourceSortingOn() {
test(new String[] {
"goog.require('beer');",
"goog.provide('beer');"
},
new String[] {
"var beer = {};",
""
});
}
public void testSourceSortingCircularDeps1() {
args.add("--manage_closure_dependencies=true");
test(new String[] {
"goog.provide('gin'); goog.require('tonic'); var gin = {};",
"goog.provide('tonic'); goog.require('gin'); var tonic = {};",
"goog.require('gin'); goog.require('tonic');"
},
JSModule.CIRCULAR_DEPENDENCY_ERROR);
}
public void testSourceSortingCircularDeps2() {
args.add("--manage_closure_dependencies=true");
test(new String[] {
"goog.provide('roses.lime.juice');",
"goog.provide('gin'); goog.require('tonic'); var gin = {};",
"goog.provide('tonic'); goog.require('gin'); var tonic = {};",
"goog.require('gin'); goog.require('tonic');",
"goog.provide('gimlet');" +
" goog.require('gin'); goog.require('roses.lime.juice');"
},
JSModule.CIRCULAR_DEPENDENCY_ERROR);
}
public void testSourcePruningOn1() {
args.add("--manage_closure_dependencies=true");
test(new String[] {
"goog.require('beer');",
"goog.provide('beer');",
"goog.provide('scotch'); var x = 3;"
},
new String[] {
"var beer = {};",
""
});
}
public void testSourcePruningOn2() {
args.add("--closure_entry_point=guinness");
test(new String[] {
"goog.provide('guinness');\ngoog.require('beer');",
"goog.provide('beer');",
"goog.provide('scotch'); var x = 3;"
},
new String[] {
"var beer = {};",
"var guinness = {};"
});
}
public void testSourcePruningOn3() {
args.add("--closure_entry_point=scotch");
test(new String[] {
"goog.provide('guinness');\ngoog.require('beer');",
"goog.provide('beer');",
"goog.provide('scotch'); var x = 3;"
},
new String[] {
"var scotch = {}, x = 3;",
});
}
public void testSourcePruningOn4() {
args.add("--closure_entry_point=scotch");
args.add("--closure_entry_point=beer");
test(new String[] {
"goog.provide('guinness');\ngoog.require('beer');",
"goog.provide('beer');",
"goog.provide('scotch'); var x = 3;"
},
new String[] {
"var beer = {};",
"var scotch = {}, x = 3;",
});
}
public void testSourcePruningOn5() {
args.add("--closure_entry_point=shiraz");
test(new String[] {
"goog.provide('guinness');\ngoog.require('beer');",
"goog.provide('beer');",
"goog.provide('scotch'); var x = 3;"
},
Compiler.MISSING_ENTRY_ERROR);
}
public void testSourcePruningOn6() {
args.add("--closure_entry_point=scotch");
test(new String[] {
"goog.require('beer');",
"goog.provide('beer');",
"goog.provide('scotch'); var x = 3;"
},
new String[] {
"var beer = {};",
"",
"var scotch = {}, x = 3;",
});
}
public void testDependencySortingWhitespaceMode() {
args.add("--manage_closure_dependencies");
args.add("--compilation_level=WHITESPACE_ONLY");
test(new String[] {
"goog.require('beer');",
"goog.provide('beer');\ngoog.require('hops');",
"goog.provide('hops');",
},
new String[] {
"goog.provide('hops');",
"goog.provide('beer');\ngoog.require('hops');",
"goog.require('beer');"
});
}
public void testForwardDeclareDroppedTypes() {
args.add("--manage_closure_dependencies=true");
args.add("--warning_level=VERBOSE");
test(new String[] {
"goog.require('beer');",
"goog.provide('beer'); /** @param {Scotch} x */ function f(x) {}",
"goog.provide('Scotch'); var x = 3;"
},
new String[] {
"var beer = {}; function f() {}",
""
});
test(new String[] {
"goog.require('beer');",
"goog.provide('beer'); /** @param {Scotch} x */ function f(x) {}"
},
new String[] {
"var beer = {}; function f() {}",
""
},
RhinoErrorReporter.TYPE_PARSE_ERROR);
}
public void testOnlyClosureDependenciesEmptyEntryPoints() throws Exception {
// Prevents this from trying to load externs.zip
args.add("--use_only_custom_externs=true");
args.add("--only_closure_dependencies=true");
try {
CommandLineRunner runner = createCommandLineRunner(new String[0]);
runner.doRun();
fail("Expected FlagUsageException");
} catch (FlagUsageException e) {
assertTrue(e.getMessage(),
e.getMessage().contains("only_closure_dependencies"));
}
}
public void testOnlyClosureDependenciesOneEntryPoint() throws Exception {
args.add("--only_closure_dependencies=true");
args.add("--closure_entry_point=beer");
test(new String[] {
"goog.require('beer'); var beerRequired = 1;",
"goog.provide('beer');\ngoog.require('hops');\nvar beerProvided = 1;",
"goog.provide('hops'); var hopsProvided = 1;",
"goog.provide('scotch'); var scotchProvided = 1;",
"var includeFileWithoutProvides = 1;"
},
new String[] {
"var hops = {}, hopsProvided = 1;",
"var beer = {}, beerProvided = 1;"
});
}
public void testSourceMapExpansion1() {
args.add("--js_output_file");
args.add("/path/to/out.js");
args.add("--create_source_map=%outname%.map");
testSame("var x = 3;");
assertEquals("/path/to/out.js.map",
lastCommandLineRunner.expandSourceMapPath(
lastCompiler.getOptions(), null));
}
public void testSourceMapExpansion2() {
useModules = ModulePattern.CHAIN;
args.add("--create_source_map=%outname%.map");
args.add("--module_output_path_prefix=foo");
testSame(new String[] {"var x = 3;", "var y = 5;"});
assertEquals("foo.map",
lastCommandLineRunner.expandSourceMapPath(
lastCompiler.getOptions(), null));
}
public void testSourceMapExpansion3() {
useModules = ModulePattern.CHAIN;
args.add("--create_source_map=%outname%.map");
args.add("--module_output_path_prefix=foo_");
testSame(new String[] {"var x = 3;", "var y = 5;"});
assertEquals("foo_m0.js.map",
lastCommandLineRunner.expandSourceMapPath(
lastCompiler.getOptions(),
lastCompiler.getModuleGraph().getRootModule()));
}
public void testSourceMapFormat1() {
args.add("--js_output_file");
args.add("/path/to/out.js");
testSame("var x = 3;");
assertEquals(SourceMap.Format.DEFAULT,
lastCompiler.getOptions().sourceMapFormat);
}
public void testSourceMapFormat2() {
args.add("--js_output_file");
args.add("/path/to/out.js");
args.add("--source_map_format=V3");
testSame("var x = 3;");
assertEquals(SourceMap.Format.V3,
lastCompiler.getOptions().sourceMapFormat);
}
public void testModuleWrapperBaseNameExpansion() throws Exception {
useModules = ModulePattern.CHAIN;
args.add("--module_wrapper=m0:%s // %basename%");
testSame(new String[] {
"var x = 3;",
"var y = 4;"
});
StringBuilder builder = new StringBuilder();
lastCommandLineRunner.writeModuleOutput(
builder,
lastCompiler.getModuleGraph().getRootModule());
assertEquals("var x=3; // m0.js\n", builder.toString());
}
public void testCharSetExpansion() {
testSame("");
assertEquals("US-ASCII", lastCompiler.getOptions().outputCharset);
args.add("--charset=UTF-8");
testSame("");
assertEquals("UTF-8", lastCompiler.getOptions().outputCharset);
}
public void testChainModuleManifest() throws Exception {
useModules = ModulePattern.CHAIN;
testSame(new String[] {
"var x = 3;", "var y = 5;", "var z = 7;", "var a = 9;"});
StringBuilder builder = new StringBuilder();
lastCommandLineRunner.printModuleGraphManifestOrBundleTo(
lastCompiler.getModuleGraph(), builder, true);
assertEquals(
"{m0}\n" +
"i0\n" +
"\n" +
"{m1:m0}\n" +
"i1\n" +
"\n" +
"{m2:m1}\n" +
"i2\n" +
"\n" +
"{m3:m2}\n" +
"i3\n",
builder.toString());
}
public void testStarModuleManifest() throws Exception {
useModules = ModulePattern.STAR;
testSame(new String[] {
"var x = 3;", "var y = 5;", "var z = 7;", "var a = 9;"});
StringBuilder builder = new StringBuilder();
lastCommandLineRunner.printModuleGraphManifestOrBundleTo(
lastCompiler.getModuleGraph(), builder, true);
assertEquals(
"{m0}\n" +
"i0\n" +
"\n" +
"{m1:m0}\n" +
"i1\n" +
"\n" +
"{m2:m0}\n" +
"i2\n" +
"\n" +
"{m3:m0}\n" +
"i3\n",
builder.toString());
}
public void testVersionFlag() {
args.add("--version");
testSame("");
assertEquals(
0,
new String(errReader.toByteArray()).indexOf(
"Closure Compiler (http://code.google.com/closure/compiler)\n" +
"Version: "));
}
public void testVersionFlag2() {
lastArg = "--version";
testSame("");
assertEquals(
0,
new String(errReader.toByteArray()).indexOf(
"Closure Compiler (http://code.google.com/closure/compiler)\n" +
"Version: "));
}
public void testPrintAstFlag() {
args.add("--print_ast=true");
testSame("");
assertEquals(
"digraph AST {\n" +
" node [color=lightblue2, style=filled];\n" +
" node0 [label=\"BLOCK\"];\n" +
" node1 [label=\"SCRIPT\"];\n" +
" node0 -> node1 [weight=1];\n" +
" node1 -> RETURN [label=\"UNCOND\", " +
"fontcolor=\"red\", weight=0.01, color=\"red\"];\n" +
" node0 -> RETURN [label=\"SYN_BLOCK\", " +
"fontcolor=\"red\", weight=0.01, color=\"red\"];\n" +
" node0 -> node1 [label=\"UNCOND\", " +
"fontcolor=\"red\", weight=0.01, color=\"red\"];\n" +
"}\n\n",
new String(outReader.toByteArray()));
}
public void testSyntheticExterns() {
externs = ImmutableList.of(
SourceFile.fromCode("externs", "myVar.property;"));
test("var theirVar = {}; var myVar = {}; var yourVar = {};",
VarCheck.UNDEFINED_EXTERN_VAR_ERROR);
args.add("--jscomp_off=externsValidation");
args.add("--warning_level=VERBOSE");
test("var theirVar = {}; var myVar = {}; var yourVar = {};",
"var theirVar={},myVar={},yourVar={};");
args.add("--jscomp_off=externsValidation");
args.add("--warning_level=VERBOSE");
test("var theirVar = {}; var myVar = {}; var myVar = {};",
SyntacticScopeCreator.VAR_MULTIPLY_DECLARED_ERROR);
}
public void testGoogAssertStripping() {
args.add("--compilation_level=ADVANCED_OPTIMIZATIONS");
test("goog.asserts.assert(false)",
"");
args.add("--debug");
test("goog.asserts.assert(false)", "goog.$asserts$.$assert$(!1)");
}
public void testMissingReturnCheckOnWithVerbose() {
args.add("--warning_level=VERBOSE");
test("/** @return {number} */ function f() {f()} f();",
CheckMissingReturn.MISSING_RETURN_STATEMENT);
}
public void testGenerateExports() {
args.add("--generate_exports=true");
test("/** @export */ foo.prototype.x = function() {};",
"foo.prototype.x=function(){};"+
"goog.exportSymbol(\"foo.prototype.x\",foo.prototype.x);");
}
public void testDepreciationWithVerbose() {
args.add("--warning_level=VERBOSE");
test("/** @deprecated */ function f() {}; f()",
CheckAccessControls.DEPRECATED_NAME);
}
public void testTwoParseErrors() {
// If parse errors are reported in different files, make
// sure all of them are reported.
Compiler compiler = compile(new String[] {
"var a b;",
"var b c;"
});
assertEquals(2, compiler.getErrors().length);
}
public void testES3ByDefault() {
test("var x = f.function", RhinoErrorReporter.PARSE_ERROR);
}
public void testES5() {
args.add("--language_in=ECMASCRIPT5");
test("var x = f.function", "var x = f.function");
test("var let", "var let");
}
public void testES5Strict() {
args.add("--language_in=ECMASCRIPT5_STRICT");
test("var x = f.function", "'use strict';var x = f.function");
test("var let", RhinoErrorReporter.PARSE_ERROR);
}
public void testES5StrictUseStrict() {
args.add("--language_in=ECMASCRIPT5_STRICT");
Compiler compiler = compile(new String[] {"var x = f.function"});
String outputSource = compiler.toSource();
assertEquals("'use strict'", outputSource.substring(0, 12));
}
public void testES5StrictUseStrictMultipleInputs() {
args.add("--language_in=ECMASCRIPT5_STRICT");
Compiler compiler = compile(new String[] {"var x = f.function",
"var y = f.function", "var z = f.function"});
String outputSource = compiler.toSource();
assertEquals("'use strict'", outputSource.substring(0, 12));
assertEquals(outputSource.substring(13).indexOf("'use strict'"), -1);
}
public void testWithKeywordDefault() {
test("var x = {}; with (x) {}", ControlStructureCheck.USE_OF_WITH);
}
public void testWithKeywordWithEs5ChecksOff() {
args.add("--jscomp_off=es5Strict");
testSame("var x = {}; with (x) {}");
}
public void testNoSrCFilesWithManifest() throws IOException {
args.add("--use_only_custom_externs=true");
args.add("--output_manifest=test.MF");
CommandLineRunner runner = createCommandLineRunner(new String[0]);
String expectedMessage = "";
try {
runner.doRun();
} catch (FlagUsageException e) {
expectedMessage = e.getMessage();
}
assertEquals(expectedMessage, "Bad --js flag. " +
"Manifest files cannot be generated when the input is from stdin.");
}
public void testTransformAMD() {
args.add("--transform_amd_modules");
test("define({test: 1})", "exports = {test: 1}");
}
public void testProcessCJS() {
args.add("--process_common_js_modules");
args.add("--common_js_entry_module=foo/bar");
setFilename(0, "foo/bar.js");
test("exports.test = 1",
"var module$foo$bar={test:1}; " +
"module$foo$bar.module$exports && " +
"(module$foo$bar=module$foo$bar.module$exports)");
}
public void testTransformAMDAndProcessCJS() {
args.add("--transform_amd_modules");
args.add("--process_common_js_modules");
args.add("--common_js_entry_module=foo/bar");
setFilename(0, "foo/bar.js");
test("define({foo: 1})",
"var module$foo$bar={}, module$foo$bar={foo:1}; " +
"module$foo$bar.module$exports && " +
"(module$foo$bar=module$foo$bar.module$exports)");
}
/* Helper functions */
private void testSame(String original) {
testSame(new String[] { original });
}
private void testSame(String[] original) {
test(original, original);
}
private void test(String original, String compiled) {
test(new String[] { original }, new String[] { compiled });
}
/**
* Asserts that when compiling with the given compiler options,
* {@code original} is transformed into {@code compiled}.
*/
private void test(String[] original, String[] compiled) {
test(original, compiled, null);
}
/**
* Asserts that when compiling with the given compiler options,
* {@code original} is transformed into {@code compiled}.
* If {@code warning} is non-null, we will also check if the given
* warning type was emitted.
*/
private void test(String[] original, String[] compiled,
DiagnosticType warning) {
Compiler compiler = compile(original);
if (warning == null) {
assertEquals("Expected no warnings or errors\n" +
"Errors: \n" + Joiner.on("\n").join(compiler.getErrors()) +
"Warnings: \n" + Joiner.on("\n").join(compiler.getWarnings()),
0, compiler.getErrors().length + compiler.getWarnings().length);
} else {
assertEquals(1, compiler.getWarnings().length);
assertEquals(warning, compiler.getWarnings()[0].getType());
}
Node root = compiler.getRoot().getLastChild();
if (useStringComparison) {
assertEquals(Joiner.on("").join(compiled), compiler.toSource());
} else {
Node expectedRoot = parse(compiled);
String explanation = expectedRoot.checkTreeEquals(root);
assertNull("\nExpected: " + compiler.toSource(expectedRoot) +
"\nResult: " + compiler.toSource(root) +
"\n" + explanation, explanation);
}
}
/**
* Asserts that when compiling, there is an error or warning.
*/
private void test(String original, DiagnosticType warning) {
test(new String[] { original }, warning);
}
private void test(String original, String expected, DiagnosticType warning) {
test(new String[] { original }, new String[] { expected }, warning);
}
/**
* Asserts that when compiling, there is an error or warning.
*/
private void test(String[] original, DiagnosticType warning) {
Compiler compiler = compile(original);
assertEquals("Expected exactly one warning or error " +
"Errors: \n" + Joiner.on("\n").join(compiler.getErrors()) +
"Warnings: \n" + Joiner.on("\n").join(compiler.getWarnings()),
1, compiler.getErrors().length + compiler.getWarnings().length);
assertTrue(exitCodes.size() > 0);
int lastExitCode = exitCodes.get(exitCodes.size() - 1);
if (compiler.getErrors().length > 0) {
assertEquals(1, compiler.getErrors().length);
assertEquals(warning, compiler.getErrors()[0].getType());
assertEquals(1, lastExitCode);
} else {
assertEquals(1, compiler.getWarnings().length);
assertEquals(warning, compiler.getWarnings()[0].getType());
assertEquals(0, lastExitCode);
}
}
private CommandLineRunner createCommandLineRunner(String[] original) {
for (int i = 0; i < original.length; i++) {
args.add("--js");
args.add("/path/to/input" + i + ".js");
if (useModules == ModulePattern.CHAIN) {
args.add("--module");
args.add("m" + i + ":1" + (i > 0 ? (":m" + (i - 1)) : ""));
} else if (useModules == ModulePattern.STAR) {
args.add("--module");
args.add("m" + i + ":1" + (i > 0 ? ":m0" : ""));
}
}
if (lastArg != null) {
args.add(lastArg);
}
String[] argStrings = args.toArray(new String[] {});
return new CommandLineRunner(
argStrings,
new PrintStream(outReader),
new PrintStream(errReader));
}
private Compiler compile(String[] original) {
CommandLineRunner runner = createCommandLineRunner(original);
assertTrue(runner.shouldRunCompiler());
Supplier<List<SourceFile>> inputsSupplier = null;
Supplier<List<JSModule>> modulesSupplier = null;
if (useModules == ModulePattern.NONE) {
List<SourceFile> inputs = Lists.newArrayList();
for (int i = 0; i < original.length; i++) {
inputs.add(SourceFile.fromCode(getFilename(i), original[i]));
}
inputsSupplier = Suppliers.ofInstance(inputs);
} else if (useModules == ModulePattern.STAR) {
modulesSupplier = Suppliers.<List<JSModule>>ofInstance(
Lists.<JSModule>newArrayList(
CompilerTestCase.createModuleStar(original)));
} else if (useModules == ModulePattern.CHAIN) {
modulesSupplier = Suppliers.<List<JSModule>>ofInstance(
Lists.<JSModule>newArrayList(
CompilerTestCase.createModuleChain(original)));
} else {
throw new IllegalArgumentException("Unknown module type: " + useModules);
}
runner.enableTestMode(
Suppliers.<List<SourceFile>>ofInstance(externs),
inputsSupplier,
modulesSupplier,
new Function<Integer, Boolean>() {
@Override
public Boolean apply(Integer code) {
return exitCodes.add(code);
}
});
runner.run();
lastCompiler = runner.getCompiler();
lastCommandLineRunner = runner;
return lastCompiler;
}
private Node parse(String[] original) {
String[] argStrings = args.toArray(new String[] {});
CommandLineRunner runner = new CommandLineRunner(argStrings);
Compiler compiler = runner.createCompiler();
List<SourceFile> inputs = Lists.newArrayList();
for (int i = 0; i < original.length; i++) {
inputs.add(SourceFile.fromCode(getFilename(i), original[i]));
}
CompilerOptions options = new CompilerOptions();
// ECMASCRIPT5 is the most forgiving.
options.setLanguageIn(LanguageMode.ECMASCRIPT5);
compiler.init(externs, inputs, options);
Node all = compiler.parseInputs();
Preconditions.checkState(compiler.getErrorCount() == 0);
Preconditions.checkNotNull(all);
Node n = all.getLastChild();
return n;
}
private void setFilename(int i, String filename) {
this.filenames.put(i, filename);
}
private String getFilename(int i) {
if (filenames.isEmpty()) {
return "input" + i;
}
return filenames.get(i);
}
} | // You are a professional Java test case writer, please create a test case named `testDependencySortingWhitespaceMode` for the issue `Closure-703`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-703
//
// ## Issue-Title:
// Add support for --manage_closure_dependencies and --only_closure_dependencies with compilation level WHITESPACE_ONLY
//
// ## Issue-Description:
// The compiler options --manage\_closure\_dependencies and --only\_closure\_dependencies are currently ignored with compilation level WHITESPACE\_ONLY. It would be helpful for testing, if dependency management were supported for WHITESPACE\_ONLY in addition to SIMPLE\_OPTIMIZATIONS and ADVANCED\_OPTIMIZATIONS. For example, both Closure Builder and plovr automatically manage dependencies for all compilation levels.
//
// The proposed change (see attached diff) does not automatically manage dependencies, but it enables dependency management if either --manage\_closure\_dependencies or --only\_closure\_dependencies is specified, or if at least one --closure\_entry\_point is specified.
//
// The attached diff passed the JUnit tests: ant test
//
//
public void testDependencySortingWhitespaceMode() {
| 636 | 31 | 623 | test/com/google/javascript/jscomp/CommandLineRunnerTest.java | test | ```markdown
## Issue-ID: Closure-703
## Issue-Title:
Add support for --manage_closure_dependencies and --only_closure_dependencies with compilation level WHITESPACE_ONLY
## Issue-Description:
The compiler options --manage\_closure\_dependencies and --only\_closure\_dependencies are currently ignored with compilation level WHITESPACE\_ONLY. It would be helpful for testing, if dependency management were supported for WHITESPACE\_ONLY in addition to SIMPLE\_OPTIMIZATIONS and ADVANCED\_OPTIMIZATIONS. For example, both Closure Builder and plovr automatically manage dependencies for all compilation levels.
The proposed change (see attached diff) does not automatically manage dependencies, but it enables dependency management if either --manage\_closure\_dependencies or --only\_closure\_dependencies is specified, or if at least one --closure\_entry\_point is specified.
The attached diff passed the JUnit tests: ant test
```
You are a professional Java test case writer, please create a test case named `testDependencySortingWhitespaceMode` for the issue `Closure-703`, utilizing the provided issue report information and the following function signature.
```java
public void testDependencySortingWhitespaceMode() {
```
| 623 | [
"com.google.javascript.jscomp.Compiler"
] | 8288fa0343aca972f07cbb30ab15a188f39c92e29ed749c5797dffdfb4a1fb41 | public void testDependencySortingWhitespaceMode() | // You are a professional Java test case writer, please create a test case named `testDependencySortingWhitespaceMode` for the issue `Closure-703`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-703
//
// ## Issue-Title:
// Add support for --manage_closure_dependencies and --only_closure_dependencies with compilation level WHITESPACE_ONLY
//
// ## Issue-Description:
// The compiler options --manage\_closure\_dependencies and --only\_closure\_dependencies are currently ignored with compilation level WHITESPACE\_ONLY. It would be helpful for testing, if dependency management were supported for WHITESPACE\_ONLY in addition to SIMPLE\_OPTIMIZATIONS and ADVANCED\_OPTIMIZATIONS. For example, both Closure Builder and plovr automatically manage dependencies for all compilation levels.
//
// The proposed change (see attached diff) does not automatically manage dependencies, but it enables dependency management if either --manage\_closure\_dependencies or --only\_closure\_dependencies is specified, or if at least one --closure\_entry\_point is specified.
//
// The attached diff passed the JUnit tests: ant test
//
//
| Closure | /*
* Copyright 2009 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import com.google.common.base.Function;
import com.google.common.base.Joiner;
import com.google.common.base.Preconditions;
import com.google.common.base.Supplier;
import com.google.common.base.Suppliers;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.Lists;
import com.google.common.collect.Maps;
import com.google.javascript.jscomp.AbstractCommandLineRunner.FlagUsageException;
import com.google.javascript.jscomp.CompilerOptions.LanguageMode;
import com.google.javascript.rhino.Node;
import junit.framework.TestCase;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.PrintStream;
import java.util.List;
import java.util.Map;
/**
* Tests for {@link CommandLineRunner}.
*
* @author [email protected] (Nick Santos)
*/
public class CommandLineRunnerTest extends TestCase {
private Compiler lastCompiler = null;
private CommandLineRunner lastCommandLineRunner = null;
private List<Integer> exitCodes = null;
private ByteArrayOutputStream outReader = null;
private ByteArrayOutputStream errReader = null;
private Map<Integer,String> filenames;
// If set, this will be appended to the end of the args list.
// For testing args parsing.
private String lastArg = null;
// If set to true, uses comparison by string instead of by AST.
private boolean useStringComparison = false;
private ModulePattern useModules = ModulePattern.NONE;
private enum ModulePattern {
NONE,
CHAIN,
STAR
}
private List<String> args = Lists.newArrayList();
/** Externs for the test */
private final List<SourceFile> DEFAULT_EXTERNS = ImmutableList.of(
SourceFile.fromCode("externs",
"var arguments;"
+ "/**\n"
+ " * @constructor\n"
+ " * @param {...*} var_args\n"
+ " */\n"
+ "function Function(var_args) {}\n"
+ "/**\n"
+ " * @param {...*} var_args\n"
+ " * @return {*}\n"
+ " */\n"
+ "Function.prototype.call = function(var_args) {};"
+ "/**\n"
+ " * @constructor\n"
+ " * @param {...*} var_args\n"
+ " * @return {!Array}\n"
+ " */\n"
+ "function Array(var_args) {}"
+ "/**\n"
+ " * @param {*=} opt_begin\n"
+ " * @param {*=} opt_end\n"
+ " * @return {!Array}\n"
+ " * @this {Object}\n"
+ " */\n"
+ "Array.prototype.slice = function(opt_begin, opt_end) {};"
+ "/** @constructor */ function Window() {}\n"
+ "/** @type {string} */ Window.prototype.name;\n"
+ "/** @type {Window} */ var window;"
+ "/** @constructor */ function Element() {}"
+ "Element.prototype.offsetWidth;"
+ "/** @nosideeffects */ function noSideEffects() {}")
);
private List<SourceFile> externs;
@Override
public void setUp() throws Exception {
super.setUp();
externs = DEFAULT_EXTERNS;
filenames = Maps.newHashMap();
lastCompiler = null;
lastArg = null;
outReader = new ByteArrayOutputStream();
errReader = new ByteArrayOutputStream();
useStringComparison = false;
useModules = ModulePattern.NONE;
args.clear();
exitCodes = Lists.newArrayList();
}
@Override
public void tearDown() throws Exception {
super.tearDown();
}
public void testWarningGuardOrdering1() {
args.add("--jscomp_error=globalThis");
args.add("--jscomp_off=globalThis");
testSame("function f() { this.a = 3; }");
}
public void testWarningGuardOrdering2() {
args.add("--jscomp_off=globalThis");
args.add("--jscomp_error=globalThis");
test("function f() { this.a = 3; }", CheckGlobalThis.GLOBAL_THIS);
}
public void testWarningGuardOrdering3() {
args.add("--jscomp_warning=globalThis");
args.add("--jscomp_off=globalThis");
testSame("function f() { this.a = 3; }");
}
public void testWarningGuardOrdering4() {
args.add("--jscomp_off=globalThis");
args.add("--jscomp_warning=globalThis");
test("function f() { this.a = 3; }", CheckGlobalThis.GLOBAL_THIS);
}
public void testCheckGlobalThisOffByDefault() {
testSame("function f() { this.a = 3; }");
}
public void testCheckGlobalThisOnWithAdvancedMode() {
args.add("--compilation_level=ADVANCED_OPTIMIZATIONS");
test("function f() { this.a = 3; }", CheckGlobalThis.GLOBAL_THIS);
}
public void testCheckGlobalThisOnWithErrorFlag() {
args.add("--jscomp_error=globalThis");
test("function f() { this.a = 3; }", CheckGlobalThis.GLOBAL_THIS);
}
public void testCheckGlobalThisOff() {
args.add("--warning_level=VERBOSE");
args.add("--jscomp_off=globalThis");
testSame("function f() { this.a = 3; }");
}
public void testTypeCheckingOffByDefault() {
test("function f(x) { return x; } f();",
"function f(a) { return a; } f();");
}
public void testReflectedMethods() {
args.add("--compilation_level=ADVANCED_OPTIMIZATIONS");
test(
"/** @constructor */" +
"function Foo() {}" +
"Foo.prototype.handle = function(x, y) { alert(y); };" +
"var x = goog.reflect.object(Foo, {handle: 1});" +
"for (var i in x) { x[i].call(x); }" +
"window['Foo'] = Foo;",
"function a() {}" +
"a.prototype.a = function(e, d) { alert(d); };" +
"var b = goog.c.b(a, {a: 1}),c;" +
"for (c in b) { b[c].call(b); }" +
"window.Foo = a;");
}
public void testTypeCheckingOnWithVerbose() {
args.add("--warning_level=VERBOSE");
test("function f(x) { return x; } f();", TypeCheck.WRONG_ARGUMENT_COUNT);
}
public void testTypeParsingOffByDefault() {
testSame("/** @return {number */ function f(a) { return a; }");
}
public void testTypeParsingOnWithVerbose() {
args.add("--warning_level=VERBOSE");
test("/** @return {number */ function f(a) { return a; }",
RhinoErrorReporter.TYPE_PARSE_ERROR);
test("/** @return {n} */ function f(a) { return a; }",
RhinoErrorReporter.TYPE_PARSE_ERROR);
}
public void testTypeCheckOverride1() {
args.add("--warning_level=VERBOSE");
args.add("--jscomp_off=checkTypes");
testSame("var x = x || {}; x.f = function() {}; x.f(3);");
}
public void testTypeCheckOverride2() {
args.add("--warning_level=DEFAULT");
testSame("var x = x || {}; x.f = function() {}; x.f(3);");
args.add("--jscomp_warning=checkTypes");
test("var x = x || {}; x.f = function() {}; x.f(3);",
TypeCheck.WRONG_ARGUMENT_COUNT);
}
public void testCheckSymbolsOffForDefault() {
args.add("--warning_level=DEFAULT");
test("x = 3; var y; var y;", "x=3; var y;");
}
public void testCheckSymbolsOnForVerbose() {
args.add("--warning_level=VERBOSE");
test("x = 3;", VarCheck.UNDEFINED_VAR_ERROR);
test("var y; var y;", SyntacticScopeCreator.VAR_MULTIPLY_DECLARED_ERROR);
}
public void testCheckSymbolsOverrideForVerbose() {
args.add("--warning_level=VERBOSE");
args.add("--jscomp_off=undefinedVars");
testSame("x = 3;");
}
public void testCheckSymbolsOverrideForQuiet() {
args.add("--warning_level=QUIET");
args.add("--jscomp_error=undefinedVars");
test("x = 3;", VarCheck.UNDEFINED_VAR_ERROR);
}
public void testCheckUndefinedProperties1() {
args.add("--warning_level=VERBOSE");
args.add("--jscomp_error=missingProperties");
test("var x = {}; var y = x.bar;", TypeCheck.INEXISTENT_PROPERTY);
}
public void testCheckUndefinedProperties2() {
args.add("--warning_level=VERBOSE");
args.add("--jscomp_off=missingProperties");
test("var x = {}; var y = x.bar;", CheckGlobalNames.UNDEFINED_NAME_WARNING);
}
public void testCheckUndefinedProperties3() {
args.add("--warning_level=VERBOSE");
test("function f() {var x = {}; var y = x.bar;}",
TypeCheck.INEXISTENT_PROPERTY);
}
public void testDuplicateParams() {
test("function f(a, a) {}", RhinoErrorReporter.DUPLICATE_PARAM);
assertTrue(lastCompiler.hasHaltingErrors());
}
public void testDefineFlag() {
args.add("--define=FOO");
args.add("--define=\"BAR=5\"");
args.add("--D"); args.add("CCC");
args.add("-D"); args.add("DDD");
test("/** @define {boolean} */ var FOO = false;" +
"/** @define {number} */ var BAR = 3;" +
"/** @define {boolean} */ var CCC = false;" +
"/** @define {boolean} */ var DDD = false;",
"var FOO = !0, BAR = 5, CCC = !0, DDD = !0;");
}
public void testDefineFlag2() {
args.add("--define=FOO='x\"'");
test("/** @define {string} */ var FOO = \"a\";",
"var FOO = \"x\\\"\";");
}
public void testDefineFlag3() {
args.add("--define=FOO=\"x'\"");
test("/** @define {string} */ var FOO = \"a\";",
"var FOO = \"x'\";");
}
public void testScriptStrictModeNoWarning() {
test("'use strict';", "");
test("'no use strict';", CheckSideEffects.USELESS_CODE_ERROR);
}
public void testFunctionStrictModeNoWarning() {
test("function f() {'use strict';}", "function f() {}");
test("function f() {'no use strict';}",
CheckSideEffects.USELESS_CODE_ERROR);
}
public void testQuietMode() {
args.add("--warning_level=DEFAULT");
test("/** @const \n * @const */ var x;",
RhinoErrorReporter.PARSE_ERROR);
args.add("--warning_level=QUIET");
testSame("/** @const \n * @const */ var x;");
}
public void testProcessClosurePrimitives() {
test("var goog = {}; goog.provide('goog.dom');",
"var goog = {dom:{}};");
args.add("--process_closure_primitives=false");
testSame("var goog = {}; goog.provide('goog.dom');");
}
public void testGetMsgWiring() throws Exception {
test("var goog = {}; goog.getMsg = function(x) { return x; };" +
"/** @desc A real foo. */ var MSG_FOO = goog.getMsg('foo');",
"var goog={getMsg:function(a){return a}}, " +
"MSG_FOO=goog.getMsg('foo');");
args.add("--compilation_level=ADVANCED_OPTIMIZATIONS");
test("var goog = {}; goog.getMsg = function(x) { return x; };" +
"/** @desc A real foo. */ var MSG_FOO = goog.getMsg('foo');" +
"window['foo'] = MSG_FOO;",
"window.foo = 'foo';");
}
public void testCssNameWiring() throws Exception {
test("var goog = {}; goog.getCssName = function() {};" +
"goog.setCssNameMapping = function() {};" +
"goog.setCssNameMapping({'goog': 'a', 'button': 'b'});" +
"var a = goog.getCssName('goog-button');" +
"var b = goog.getCssName('css-button');" +
"var c = goog.getCssName('goog-menu');" +
"var d = goog.getCssName('css-menu');",
"var goog = { getCssName: function() {}," +
" setCssNameMapping: function() {} }," +
" a = 'a-b'," +
" b = 'css-b'," +
" c = 'a-menu'," +
" d = 'css-menu';");
}
//////////////////////////////////////////////////////////////////////////////
// Integration tests
public void testIssue70a() {
test("function foo({}) {}", RhinoErrorReporter.PARSE_ERROR);
}
public void testIssue70b() {
test("function foo([]) {}", RhinoErrorReporter.PARSE_ERROR);
}
public void testIssue81() {
args.add("--compilation_level=ADVANCED_OPTIMIZATIONS");
useStringComparison = true;
test("eval('1'); var x = eval; x('2');",
"eval(\"1\");(0,eval)(\"2\");");
}
public void testIssue115() {
args.add("--compilation_level=SIMPLE_OPTIMIZATIONS");
args.add("--jscomp_off=es5Strict");
args.add("--warning_level=VERBOSE");
test("function f() { " +
" var arguments = Array.prototype.slice.call(arguments, 0);" +
" return arguments[0]; " +
"}",
"function f() { " +
" arguments = Array.prototype.slice.call(arguments, 0);" +
" return arguments[0]; " +
"}");
}
public void testIssue297() {
args.add("--compilation_level=SIMPLE_OPTIMIZATIONS");
test("function f(p) {" +
" var x;" +
" return ((x=p.id) && (x=parseInt(x.substr(1))) && x>0);" +
"}",
"function f(b) {" +
" var a;" +
" return ((a=b.id) && (a=parseInt(a.substr(1))) && 0<a);" +
"}");
}
public void testHiddenSideEffect() {
args.add("--compilation_level=ADVANCED_OPTIMIZATIONS");
test("element.offsetWidth;",
"element.offsetWidth", CheckSideEffects.USELESS_CODE_ERROR);
}
public void testIssue504() {
args.add("--compilation_level=ADVANCED_OPTIMIZATIONS");
test("void function() { alert('hi'); }();",
"alert('hi');void 0", CheckSideEffects.USELESS_CODE_ERROR);
}
public void testIssue601() {
args.add("--compilation_level=WHITESPACE_ONLY");
test("function f() { return '\\v' == 'v'; } window['f'] = f;",
"function f(){return'\\v'=='v'}window['f']=f");
}
public void testIssue601b() {
args.add("--compilation_level=ADVANCED_OPTIMIZATIONS");
test("function f() { return '\\v' == 'v'; } window['f'] = f;",
"window.f=function(){return'\\v'=='v'}");
}
public void testIssue601c() {
args.add("--compilation_level=ADVANCED_OPTIMIZATIONS");
test("function f() { return '\\u000B' == 'v'; } window['f'] = f;",
"window.f=function(){return'\\u000B'=='v'}");
}
public void testDebugFlag1() {
args.add("--compilation_level=SIMPLE_OPTIMIZATIONS");
args.add("--debug=false");
test("function foo(a) {}",
"function foo() {}");
}
public void testDebugFlag2() {
args.add("--compilation_level=SIMPLE_OPTIMIZATIONS");
args.add("--debug=true");
test("function foo(a) {alert(a)}",
"function foo($a$$) {alert($a$$)}");
}
public void testDebugFlag3() {
args.add("--compilation_level=ADVANCED_OPTIMIZATIONS");
args.add("--warning_level=QUIET");
args.add("--debug=false");
test("function Foo() {}" +
"Foo.x = 1;" +
"function f() {throw new Foo().x;} f();",
"throw (new function() {}).a;");
}
public void testDebugFlag4() {
args.add("--compilation_level=ADVANCED_OPTIMIZATIONS");
args.add("--warning_level=QUIET");
args.add("--debug=true");
test("function Foo() {}" +
"Foo.x = 1;" +
"function f() {throw new Foo().x;} f();",
"throw (new function Foo() {}).$x$;");
}
public void testBooleanFlag1() {
args.add("--compilation_level=SIMPLE_OPTIMIZATIONS");
args.add("--debug");
test("function foo(a) {alert(a)}",
"function foo($a$$) {alert($a$$)}");
}
public void testBooleanFlag2() {
args.add("--debug");
args.add("--compilation_level=SIMPLE_OPTIMIZATIONS");
test("function foo(a) {alert(a)}",
"function foo($a$$) {alert($a$$)}");
}
public void testHelpFlag() {
args.add("--help");
assertFalse(
createCommandLineRunner(
new String[] {"function f() {}"}).shouldRunCompiler());
}
public void testExternsLifting1() throws Exception{
String code = "/** @externs */ function f() {}";
test(new String[] {code},
new String[] {});
assertEquals(2, lastCompiler.getExternsForTesting().size());
CompilerInput extern = lastCompiler.getExternsForTesting().get(1);
assertNull(extern.getModule());
assertTrue(extern.isExtern());
assertEquals(code, extern.getCode());
assertEquals(1, lastCompiler.getInputsForTesting().size());
CompilerInput input = lastCompiler.getInputsForTesting().get(0);
assertNotNull(input.getModule());
assertFalse(input.isExtern());
assertEquals("", input.getCode());
}
public void testExternsLifting2() {
args.add("--warning_level=VERBOSE");
test(new String[] {"/** @externs */ function f() {}", "f(3);"},
new String[] {"f(3);"},
TypeCheck.WRONG_ARGUMENT_COUNT);
}
public void testSourceSortingOff() {
args.add("--compilation_level=WHITESPACE_ONLY");
testSame(
new String[] {
"goog.require('beer');",
"goog.provide('beer');"
});
}
public void testSourceSortingOn() {
test(new String[] {
"goog.require('beer');",
"goog.provide('beer');"
},
new String[] {
"var beer = {};",
""
});
}
public void testSourceSortingCircularDeps1() {
args.add("--manage_closure_dependencies=true");
test(new String[] {
"goog.provide('gin'); goog.require('tonic'); var gin = {};",
"goog.provide('tonic'); goog.require('gin'); var tonic = {};",
"goog.require('gin'); goog.require('tonic');"
},
JSModule.CIRCULAR_DEPENDENCY_ERROR);
}
public void testSourceSortingCircularDeps2() {
args.add("--manage_closure_dependencies=true");
test(new String[] {
"goog.provide('roses.lime.juice');",
"goog.provide('gin'); goog.require('tonic'); var gin = {};",
"goog.provide('tonic'); goog.require('gin'); var tonic = {};",
"goog.require('gin'); goog.require('tonic');",
"goog.provide('gimlet');" +
" goog.require('gin'); goog.require('roses.lime.juice');"
},
JSModule.CIRCULAR_DEPENDENCY_ERROR);
}
public void testSourcePruningOn1() {
args.add("--manage_closure_dependencies=true");
test(new String[] {
"goog.require('beer');",
"goog.provide('beer');",
"goog.provide('scotch'); var x = 3;"
},
new String[] {
"var beer = {};",
""
});
}
public void testSourcePruningOn2() {
args.add("--closure_entry_point=guinness");
test(new String[] {
"goog.provide('guinness');\ngoog.require('beer');",
"goog.provide('beer');",
"goog.provide('scotch'); var x = 3;"
},
new String[] {
"var beer = {};",
"var guinness = {};"
});
}
public void testSourcePruningOn3() {
args.add("--closure_entry_point=scotch");
test(new String[] {
"goog.provide('guinness');\ngoog.require('beer');",
"goog.provide('beer');",
"goog.provide('scotch'); var x = 3;"
},
new String[] {
"var scotch = {}, x = 3;",
});
}
public void testSourcePruningOn4() {
args.add("--closure_entry_point=scotch");
args.add("--closure_entry_point=beer");
test(new String[] {
"goog.provide('guinness');\ngoog.require('beer');",
"goog.provide('beer');",
"goog.provide('scotch'); var x = 3;"
},
new String[] {
"var beer = {};",
"var scotch = {}, x = 3;",
});
}
public void testSourcePruningOn5() {
args.add("--closure_entry_point=shiraz");
test(new String[] {
"goog.provide('guinness');\ngoog.require('beer');",
"goog.provide('beer');",
"goog.provide('scotch'); var x = 3;"
},
Compiler.MISSING_ENTRY_ERROR);
}
public void testSourcePruningOn6() {
args.add("--closure_entry_point=scotch");
test(new String[] {
"goog.require('beer');",
"goog.provide('beer');",
"goog.provide('scotch'); var x = 3;"
},
new String[] {
"var beer = {};",
"",
"var scotch = {}, x = 3;",
});
}
public void testDependencySortingWhitespaceMode() {
args.add("--manage_closure_dependencies");
args.add("--compilation_level=WHITESPACE_ONLY");
test(new String[] {
"goog.require('beer');",
"goog.provide('beer');\ngoog.require('hops');",
"goog.provide('hops');",
},
new String[] {
"goog.provide('hops');",
"goog.provide('beer');\ngoog.require('hops');",
"goog.require('beer');"
});
}
public void testForwardDeclareDroppedTypes() {
args.add("--manage_closure_dependencies=true");
args.add("--warning_level=VERBOSE");
test(new String[] {
"goog.require('beer');",
"goog.provide('beer'); /** @param {Scotch} x */ function f(x) {}",
"goog.provide('Scotch'); var x = 3;"
},
new String[] {
"var beer = {}; function f() {}",
""
});
test(new String[] {
"goog.require('beer');",
"goog.provide('beer'); /** @param {Scotch} x */ function f(x) {}"
},
new String[] {
"var beer = {}; function f() {}",
""
},
RhinoErrorReporter.TYPE_PARSE_ERROR);
}
public void testOnlyClosureDependenciesEmptyEntryPoints() throws Exception {
// Prevents this from trying to load externs.zip
args.add("--use_only_custom_externs=true");
args.add("--only_closure_dependencies=true");
try {
CommandLineRunner runner = createCommandLineRunner(new String[0]);
runner.doRun();
fail("Expected FlagUsageException");
} catch (FlagUsageException e) {
assertTrue(e.getMessage(),
e.getMessage().contains("only_closure_dependencies"));
}
}
public void testOnlyClosureDependenciesOneEntryPoint() throws Exception {
args.add("--only_closure_dependencies=true");
args.add("--closure_entry_point=beer");
test(new String[] {
"goog.require('beer'); var beerRequired = 1;",
"goog.provide('beer');\ngoog.require('hops');\nvar beerProvided = 1;",
"goog.provide('hops'); var hopsProvided = 1;",
"goog.provide('scotch'); var scotchProvided = 1;",
"var includeFileWithoutProvides = 1;"
},
new String[] {
"var hops = {}, hopsProvided = 1;",
"var beer = {}, beerProvided = 1;"
});
}
public void testSourceMapExpansion1() {
args.add("--js_output_file");
args.add("/path/to/out.js");
args.add("--create_source_map=%outname%.map");
testSame("var x = 3;");
assertEquals("/path/to/out.js.map",
lastCommandLineRunner.expandSourceMapPath(
lastCompiler.getOptions(), null));
}
public void testSourceMapExpansion2() {
useModules = ModulePattern.CHAIN;
args.add("--create_source_map=%outname%.map");
args.add("--module_output_path_prefix=foo");
testSame(new String[] {"var x = 3;", "var y = 5;"});
assertEquals("foo.map",
lastCommandLineRunner.expandSourceMapPath(
lastCompiler.getOptions(), null));
}
public void testSourceMapExpansion3() {
useModules = ModulePattern.CHAIN;
args.add("--create_source_map=%outname%.map");
args.add("--module_output_path_prefix=foo_");
testSame(new String[] {"var x = 3;", "var y = 5;"});
assertEquals("foo_m0.js.map",
lastCommandLineRunner.expandSourceMapPath(
lastCompiler.getOptions(),
lastCompiler.getModuleGraph().getRootModule()));
}
public void testSourceMapFormat1() {
args.add("--js_output_file");
args.add("/path/to/out.js");
testSame("var x = 3;");
assertEquals(SourceMap.Format.DEFAULT,
lastCompiler.getOptions().sourceMapFormat);
}
public void testSourceMapFormat2() {
args.add("--js_output_file");
args.add("/path/to/out.js");
args.add("--source_map_format=V3");
testSame("var x = 3;");
assertEquals(SourceMap.Format.V3,
lastCompiler.getOptions().sourceMapFormat);
}
public void testModuleWrapperBaseNameExpansion() throws Exception {
useModules = ModulePattern.CHAIN;
args.add("--module_wrapper=m0:%s // %basename%");
testSame(new String[] {
"var x = 3;",
"var y = 4;"
});
StringBuilder builder = new StringBuilder();
lastCommandLineRunner.writeModuleOutput(
builder,
lastCompiler.getModuleGraph().getRootModule());
assertEquals("var x=3; // m0.js\n", builder.toString());
}
public void testCharSetExpansion() {
testSame("");
assertEquals("US-ASCII", lastCompiler.getOptions().outputCharset);
args.add("--charset=UTF-8");
testSame("");
assertEquals("UTF-8", lastCompiler.getOptions().outputCharset);
}
public void testChainModuleManifest() throws Exception {
useModules = ModulePattern.CHAIN;
testSame(new String[] {
"var x = 3;", "var y = 5;", "var z = 7;", "var a = 9;"});
StringBuilder builder = new StringBuilder();
lastCommandLineRunner.printModuleGraphManifestOrBundleTo(
lastCompiler.getModuleGraph(), builder, true);
assertEquals(
"{m0}\n" +
"i0\n" +
"\n" +
"{m1:m0}\n" +
"i1\n" +
"\n" +
"{m2:m1}\n" +
"i2\n" +
"\n" +
"{m3:m2}\n" +
"i3\n",
builder.toString());
}
public void testStarModuleManifest() throws Exception {
useModules = ModulePattern.STAR;
testSame(new String[] {
"var x = 3;", "var y = 5;", "var z = 7;", "var a = 9;"});
StringBuilder builder = new StringBuilder();
lastCommandLineRunner.printModuleGraphManifestOrBundleTo(
lastCompiler.getModuleGraph(), builder, true);
assertEquals(
"{m0}\n" +
"i0\n" +
"\n" +
"{m1:m0}\n" +
"i1\n" +
"\n" +
"{m2:m0}\n" +
"i2\n" +
"\n" +
"{m3:m0}\n" +
"i3\n",
builder.toString());
}
public void testVersionFlag() {
args.add("--version");
testSame("");
assertEquals(
0,
new String(errReader.toByteArray()).indexOf(
"Closure Compiler (http://code.google.com/closure/compiler)\n" +
"Version: "));
}
public void testVersionFlag2() {
lastArg = "--version";
testSame("");
assertEquals(
0,
new String(errReader.toByteArray()).indexOf(
"Closure Compiler (http://code.google.com/closure/compiler)\n" +
"Version: "));
}
public void testPrintAstFlag() {
args.add("--print_ast=true");
testSame("");
assertEquals(
"digraph AST {\n" +
" node [color=lightblue2, style=filled];\n" +
" node0 [label=\"BLOCK\"];\n" +
" node1 [label=\"SCRIPT\"];\n" +
" node0 -> node1 [weight=1];\n" +
" node1 -> RETURN [label=\"UNCOND\", " +
"fontcolor=\"red\", weight=0.01, color=\"red\"];\n" +
" node0 -> RETURN [label=\"SYN_BLOCK\", " +
"fontcolor=\"red\", weight=0.01, color=\"red\"];\n" +
" node0 -> node1 [label=\"UNCOND\", " +
"fontcolor=\"red\", weight=0.01, color=\"red\"];\n" +
"}\n\n",
new String(outReader.toByteArray()));
}
public void testSyntheticExterns() {
externs = ImmutableList.of(
SourceFile.fromCode("externs", "myVar.property;"));
test("var theirVar = {}; var myVar = {}; var yourVar = {};",
VarCheck.UNDEFINED_EXTERN_VAR_ERROR);
args.add("--jscomp_off=externsValidation");
args.add("--warning_level=VERBOSE");
test("var theirVar = {}; var myVar = {}; var yourVar = {};",
"var theirVar={},myVar={},yourVar={};");
args.add("--jscomp_off=externsValidation");
args.add("--warning_level=VERBOSE");
test("var theirVar = {}; var myVar = {}; var myVar = {};",
SyntacticScopeCreator.VAR_MULTIPLY_DECLARED_ERROR);
}
public void testGoogAssertStripping() {
args.add("--compilation_level=ADVANCED_OPTIMIZATIONS");
test("goog.asserts.assert(false)",
"");
args.add("--debug");
test("goog.asserts.assert(false)", "goog.$asserts$.$assert$(!1)");
}
public void testMissingReturnCheckOnWithVerbose() {
args.add("--warning_level=VERBOSE");
test("/** @return {number} */ function f() {f()} f();",
CheckMissingReturn.MISSING_RETURN_STATEMENT);
}
public void testGenerateExports() {
args.add("--generate_exports=true");
test("/** @export */ foo.prototype.x = function() {};",
"foo.prototype.x=function(){};"+
"goog.exportSymbol(\"foo.prototype.x\",foo.prototype.x);");
}
public void testDepreciationWithVerbose() {
args.add("--warning_level=VERBOSE");
test("/** @deprecated */ function f() {}; f()",
CheckAccessControls.DEPRECATED_NAME);
}
public void testTwoParseErrors() {
// If parse errors are reported in different files, make
// sure all of them are reported.
Compiler compiler = compile(new String[] {
"var a b;",
"var b c;"
});
assertEquals(2, compiler.getErrors().length);
}
public void testES3ByDefault() {
test("var x = f.function", RhinoErrorReporter.PARSE_ERROR);
}
public void testES5() {
args.add("--language_in=ECMASCRIPT5");
test("var x = f.function", "var x = f.function");
test("var let", "var let");
}
public void testES5Strict() {
args.add("--language_in=ECMASCRIPT5_STRICT");
test("var x = f.function", "'use strict';var x = f.function");
test("var let", RhinoErrorReporter.PARSE_ERROR);
}
public void testES5StrictUseStrict() {
args.add("--language_in=ECMASCRIPT5_STRICT");
Compiler compiler = compile(new String[] {"var x = f.function"});
String outputSource = compiler.toSource();
assertEquals("'use strict'", outputSource.substring(0, 12));
}
public void testES5StrictUseStrictMultipleInputs() {
args.add("--language_in=ECMASCRIPT5_STRICT");
Compiler compiler = compile(new String[] {"var x = f.function",
"var y = f.function", "var z = f.function"});
String outputSource = compiler.toSource();
assertEquals("'use strict'", outputSource.substring(0, 12));
assertEquals(outputSource.substring(13).indexOf("'use strict'"), -1);
}
public void testWithKeywordDefault() {
test("var x = {}; with (x) {}", ControlStructureCheck.USE_OF_WITH);
}
public void testWithKeywordWithEs5ChecksOff() {
args.add("--jscomp_off=es5Strict");
testSame("var x = {}; with (x) {}");
}
public void testNoSrCFilesWithManifest() throws IOException {
args.add("--use_only_custom_externs=true");
args.add("--output_manifest=test.MF");
CommandLineRunner runner = createCommandLineRunner(new String[0]);
String expectedMessage = "";
try {
runner.doRun();
} catch (FlagUsageException e) {
expectedMessage = e.getMessage();
}
assertEquals(expectedMessage, "Bad --js flag. " +
"Manifest files cannot be generated when the input is from stdin.");
}
public void testTransformAMD() {
args.add("--transform_amd_modules");
test("define({test: 1})", "exports = {test: 1}");
}
public void testProcessCJS() {
args.add("--process_common_js_modules");
args.add("--common_js_entry_module=foo/bar");
setFilename(0, "foo/bar.js");
test("exports.test = 1",
"var module$foo$bar={test:1}; " +
"module$foo$bar.module$exports && " +
"(module$foo$bar=module$foo$bar.module$exports)");
}
public void testTransformAMDAndProcessCJS() {
args.add("--transform_amd_modules");
args.add("--process_common_js_modules");
args.add("--common_js_entry_module=foo/bar");
setFilename(0, "foo/bar.js");
test("define({foo: 1})",
"var module$foo$bar={}, module$foo$bar={foo:1}; " +
"module$foo$bar.module$exports && " +
"(module$foo$bar=module$foo$bar.module$exports)");
}
/* Helper functions */
private void testSame(String original) {
testSame(new String[] { original });
}
private void testSame(String[] original) {
test(original, original);
}
private void test(String original, String compiled) {
test(new String[] { original }, new String[] { compiled });
}
/**
* Asserts that when compiling with the given compiler options,
* {@code original} is transformed into {@code compiled}.
*/
private void test(String[] original, String[] compiled) {
test(original, compiled, null);
}
/**
* Asserts that when compiling with the given compiler options,
* {@code original} is transformed into {@code compiled}.
* If {@code warning} is non-null, we will also check if the given
* warning type was emitted.
*/
private void test(String[] original, String[] compiled,
DiagnosticType warning) {
Compiler compiler = compile(original);
if (warning == null) {
assertEquals("Expected no warnings or errors\n" +
"Errors: \n" + Joiner.on("\n").join(compiler.getErrors()) +
"Warnings: \n" + Joiner.on("\n").join(compiler.getWarnings()),
0, compiler.getErrors().length + compiler.getWarnings().length);
} else {
assertEquals(1, compiler.getWarnings().length);
assertEquals(warning, compiler.getWarnings()[0].getType());
}
Node root = compiler.getRoot().getLastChild();
if (useStringComparison) {
assertEquals(Joiner.on("").join(compiled), compiler.toSource());
} else {
Node expectedRoot = parse(compiled);
String explanation = expectedRoot.checkTreeEquals(root);
assertNull("\nExpected: " + compiler.toSource(expectedRoot) +
"\nResult: " + compiler.toSource(root) +
"\n" + explanation, explanation);
}
}
/**
* Asserts that when compiling, there is an error or warning.
*/
private void test(String original, DiagnosticType warning) {
test(new String[] { original }, warning);
}
private void test(String original, String expected, DiagnosticType warning) {
test(new String[] { original }, new String[] { expected }, warning);
}
/**
* Asserts that when compiling, there is an error or warning.
*/
private void test(String[] original, DiagnosticType warning) {
Compiler compiler = compile(original);
assertEquals("Expected exactly one warning or error " +
"Errors: \n" + Joiner.on("\n").join(compiler.getErrors()) +
"Warnings: \n" + Joiner.on("\n").join(compiler.getWarnings()),
1, compiler.getErrors().length + compiler.getWarnings().length);
assertTrue(exitCodes.size() > 0);
int lastExitCode = exitCodes.get(exitCodes.size() - 1);
if (compiler.getErrors().length > 0) {
assertEquals(1, compiler.getErrors().length);
assertEquals(warning, compiler.getErrors()[0].getType());
assertEquals(1, lastExitCode);
} else {
assertEquals(1, compiler.getWarnings().length);
assertEquals(warning, compiler.getWarnings()[0].getType());
assertEquals(0, lastExitCode);
}
}
private CommandLineRunner createCommandLineRunner(String[] original) {
for (int i = 0; i < original.length; i++) {
args.add("--js");
args.add("/path/to/input" + i + ".js");
if (useModules == ModulePattern.CHAIN) {
args.add("--module");
args.add("m" + i + ":1" + (i > 0 ? (":m" + (i - 1)) : ""));
} else if (useModules == ModulePattern.STAR) {
args.add("--module");
args.add("m" + i + ":1" + (i > 0 ? ":m0" : ""));
}
}
if (lastArg != null) {
args.add(lastArg);
}
String[] argStrings = args.toArray(new String[] {});
return new CommandLineRunner(
argStrings,
new PrintStream(outReader),
new PrintStream(errReader));
}
private Compiler compile(String[] original) {
CommandLineRunner runner = createCommandLineRunner(original);
assertTrue(runner.shouldRunCompiler());
Supplier<List<SourceFile>> inputsSupplier = null;
Supplier<List<JSModule>> modulesSupplier = null;
if (useModules == ModulePattern.NONE) {
List<SourceFile> inputs = Lists.newArrayList();
for (int i = 0; i < original.length; i++) {
inputs.add(SourceFile.fromCode(getFilename(i), original[i]));
}
inputsSupplier = Suppliers.ofInstance(inputs);
} else if (useModules == ModulePattern.STAR) {
modulesSupplier = Suppliers.<List<JSModule>>ofInstance(
Lists.<JSModule>newArrayList(
CompilerTestCase.createModuleStar(original)));
} else if (useModules == ModulePattern.CHAIN) {
modulesSupplier = Suppliers.<List<JSModule>>ofInstance(
Lists.<JSModule>newArrayList(
CompilerTestCase.createModuleChain(original)));
} else {
throw new IllegalArgumentException("Unknown module type: " + useModules);
}
runner.enableTestMode(
Suppliers.<List<SourceFile>>ofInstance(externs),
inputsSupplier,
modulesSupplier,
new Function<Integer, Boolean>() {
@Override
public Boolean apply(Integer code) {
return exitCodes.add(code);
}
});
runner.run();
lastCompiler = runner.getCompiler();
lastCommandLineRunner = runner;
return lastCompiler;
}
private Node parse(String[] original) {
String[] argStrings = args.toArray(new String[] {});
CommandLineRunner runner = new CommandLineRunner(argStrings);
Compiler compiler = runner.createCompiler();
List<SourceFile> inputs = Lists.newArrayList();
for (int i = 0; i < original.length; i++) {
inputs.add(SourceFile.fromCode(getFilename(i), original[i]));
}
CompilerOptions options = new CompilerOptions();
// ECMASCRIPT5 is the most forgiving.
options.setLanguageIn(LanguageMode.ECMASCRIPT5);
compiler.init(externs, inputs, options);
Node all = compiler.parseInputs();
Preconditions.checkState(compiler.getErrorCount() == 0);
Preconditions.checkNotNull(all);
Node n = all.getLastChild();
return n;
}
private void setFilename(int i, String filename) {
this.filenames.put(i, filename);
}
private String getFilename(int i) {
if (filenames.isEmpty()) {
return "input" + i;
}
return filenames.get(i);
}
} |
||
public void testCodec98NPE() throws Exception {
byte[] codec98 = StringUtils.getBytesUtf8(Base64TestData.CODEC_98_NPE);
ByteArrayInputStream data = new ByteArrayInputStream(codec98);
Base64InputStream stream = new Base64InputStream(data);
// This line causes an NPE in commons-codec-1.4.jar:
byte[] decodedBytes = Base64TestData.streamToBytes(stream, new byte[1024]);
String decoded = StringUtils.newStringUtf8(decodedBytes);
assertEquals(
"codec-98 NPE Base64InputStream", Base64TestData.CODEC_98_NPE_DECODED, decoded
);
} | org.apache.commons.codec.binary.Base64InputStreamTest::testCodec98NPE | src/test/org/apache/commons/codec/binary/Base64InputStreamTest.java | 66 | src/test/org/apache/commons/codec/binary/Base64InputStreamTest.java | testCodec98NPE | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.codec.binary;
import java.io.ByteArrayInputStream;
import java.io.InputStream;
import java.util.Arrays;
import junit.framework.TestCase;
/**
* @author Apache Software Foundation
* @version $Id $
* @since 1.4
*/
public class Base64InputStreamTest extends TestCase {
private final static byte[] CRLF = {(byte) '\r', (byte) '\n'};
private final static byte[] LF = {(byte) '\n'};
private static final String STRING_FIXTURE = "Hello World";
/**
* Construct a new instance of this test case.
*
* @param name
* Name of the test case
*/
public Base64InputStreamTest(String name) {
super(name);
}
/**
* Test the Base64InputStream implementation against the special NPE inducing input
* identified in the CODEC-98 bug.
*
* @throws Exception for some failure scenarios.
*/
public void testCodec98NPE() throws Exception {
byte[] codec98 = StringUtils.getBytesUtf8(Base64TestData.CODEC_98_NPE);
ByteArrayInputStream data = new ByteArrayInputStream(codec98);
Base64InputStream stream = new Base64InputStream(data);
// This line causes an NPE in commons-codec-1.4.jar:
byte[] decodedBytes = Base64TestData.streamToBytes(stream, new byte[1024]);
String decoded = StringUtils.newStringUtf8(decodedBytes);
assertEquals(
"codec-98 NPE Base64InputStream", Base64TestData.CODEC_98_NPE_DECODED, decoded
);
}
/**
* Tests the Base64InputStream implementation against empty input.
*
* @throws Exception
* for some failure scenarios.
*/
public void testBase64EmptyInputStreamMimeChuckSize() throws Exception {
testBase64EmptyInputStream(Base64.MIME_CHUNK_SIZE);
}
/**
* Tests the Base64InputStream implementation against empty input.
*
* @throws Exception
* for some failure scenarios.
*/
public void testBase64EmptyInputStreamPemChuckSize() throws Exception {
testBase64EmptyInputStream(Base64.PEM_CHUNK_SIZE);
}
private void testBase64EmptyInputStream(int chuckSize) throws Exception {
byte[] emptyEncoded = new byte[0];
byte[] emptyDecoded = new byte[0];
testByteByByte(emptyEncoded, emptyDecoded, chuckSize, CRLF);
testByChunk(emptyEncoded, emptyDecoded, chuckSize, CRLF);
}
/**
* Tests the Base64InputStream implementation.
*
* @throws Exception
* for some failure scenarios.
*/
public void testBase64InputStreamByChunk() throws Exception {
// Hello World test.
byte[] encoded = StringUtils.getBytesUtf8("SGVsbG8gV29ybGQ=\r\n");
byte[] decoded = StringUtils.getBytesUtf8(STRING_FIXTURE);
testByChunk(encoded, decoded, Base64.MIME_CHUNK_SIZE, CRLF);
// Single Byte test.
encoded = StringUtils.getBytesUtf8("AA==\r\n");
decoded = new byte[]{(byte) 0};
testByChunk(encoded, decoded, Base64.MIME_CHUNK_SIZE, CRLF);
// OpenSSL interop test.
encoded = StringUtils.getBytesUtf8(Base64TestData.ENCODED_64_CHARS_PER_LINE);
decoded = Base64TestData.DECODED;
testByChunk(encoded, decoded, Base64.PEM_CHUNK_SIZE, LF);
// Single Line test.
String singleLine = Base64TestData.ENCODED_64_CHARS_PER_LINE.replaceAll("\n", "");
encoded = StringUtils.getBytesUtf8(singleLine);
decoded = Base64TestData.DECODED;
testByChunk(encoded, decoded, 0, LF);
// test random data of sizes 0 thru 150
for (int i = 0; i <= 150; i++) {
byte[][] randomData = Base64TestData.randomData(i, false);
encoded = randomData[1];
decoded = randomData[0];
testByChunk(encoded, decoded, 0, LF);
}
}
/**
* Tests the Base64InputStream implementation.
*
* @throws Exception
* for some failure scenarios.
*/
public void testBase64InputStreamByteByByte() throws Exception {
// Hello World test.
byte[] encoded = StringUtils.getBytesUtf8("SGVsbG8gV29ybGQ=\r\n");
byte[] decoded = StringUtils.getBytesUtf8(STRING_FIXTURE);
testByteByByte(encoded, decoded, Base64.MIME_CHUNK_SIZE, CRLF);
// Single Byte test.
encoded = StringUtils.getBytesUtf8("AA==\r\n");
decoded = new byte[]{(byte) 0};
testByteByByte(encoded, decoded, Base64.MIME_CHUNK_SIZE, CRLF);
// OpenSSL interop test.
encoded = StringUtils.getBytesUtf8(Base64TestData.ENCODED_64_CHARS_PER_LINE);
decoded = Base64TestData.DECODED;
testByteByByte(encoded, decoded, Base64.PEM_CHUNK_SIZE, LF);
// Single Line test.
String singleLine = Base64TestData.ENCODED_64_CHARS_PER_LINE.replaceAll("\n", "");
encoded = StringUtils.getBytesUtf8(singleLine);
decoded = Base64TestData.DECODED;
testByteByByte(encoded, decoded, 0, LF);
// test random data of sizes 0 thru 150
for (int i = 0; i <= 150; i++) {
byte[][] randomData = Base64TestData.randomData(i, false);
encoded = randomData[1];
decoded = randomData[0];
testByteByByte(encoded, decoded, 0, LF);
}
}
/**
* Tests method does three tests on the supplied data: 1. encoded ---[DECODE]--> decoded 2. decoded ---[ENCODE]-->
* encoded 3. decoded ---[WRAP-WRAP-WRAP-etc...] --> decoded
* <p/>
* By "[WRAP-WRAP-WRAP-etc...]" we mean situation where the Base64InputStream wraps itself in encode and decode mode
* over and over again.
*
* @param encoded
* base64 encoded data
* @param decoded
* the data from above, but decoded
* @param chunkSize
* chunk size (line-length) of the base64 encoded data.
* @param seperator
* Line separator in the base64 encoded data.
* @throws Exception
* Usually signifies a bug in the Base64 commons-codec implementation.
*/
private void testByChunk(byte[] encoded, byte[] decoded, int chunkSize, byte[] seperator) throws Exception {
// Start with encode.
InputStream in = new ByteArrayInputStream(decoded);
in = new Base64InputStream(in, true, chunkSize, seperator);
byte[] output = Base64TestData.streamToBytes(in);
assertEquals("EOF", -1, in.read());
assertEquals("Still EOF", -1, in.read());
assertTrue("Streaming base64 encode", Arrays.equals(output, encoded));
// Now let's try decode.
in = new ByteArrayInputStream(encoded);
in = new Base64InputStream(in);
output = Base64TestData.streamToBytes(in);
assertEquals("EOF", -1, in.read());
assertEquals("Still EOF", -1, in.read());
assertTrue("Streaming base64 decode", Arrays.equals(output, decoded));
// I always wanted to do this! (wrap encoder with decoder etc etc).
in = new ByteArrayInputStream(decoded);
for (int i = 0; i < 10; i++) {
in = new Base64InputStream(in, true, chunkSize, seperator);
in = new Base64InputStream(in, false);
}
output = Base64TestData.streamToBytes(in);
assertEquals("EOF", -1, in.read());
assertEquals("Still EOF", -1, in.read());
assertTrue("Streaming base64 wrap-wrap-wrap!", Arrays.equals(output, decoded));
}
/**
* Tests method does three tests on the supplied data: 1. encoded ---[DECODE]--> decoded 2. decoded ---[ENCODE]-->
* encoded 3. decoded ---[WRAP-WRAP-WRAP-etc...] --> decoded
* <p/>
* By "[WRAP-WRAP-WRAP-etc...]" we mean situation where the Base64InputStream wraps itself in encode and decode mode
* over and over again.
*
* @param encoded
* base64 encoded data
* @param decoded
* the data from above, but decoded
* @param chunkSize
* chunk size (line-length) of the base64 encoded data.
* @param seperator
* Line separator in the base64 encoded data.
* @throws Exception
* Usually signifies a bug in the Base64 commons-codec implementation.
*/
private void testByteByByte(byte[] encoded, byte[] decoded, int chunkSize, byte[] seperator) throws Exception {
// Start with encode.
InputStream in = new ByteArrayInputStream(decoded);
in = new Base64InputStream(in, true, chunkSize, seperator);
byte[] output = new byte[encoded.length];
for (int i = 0; i < output.length; i++) {
output[i] = (byte) in.read();
}
assertEquals("EOF", -1, in.read());
assertEquals("Still EOF", -1, in.read());
assertTrue("Streaming base64 encode", Arrays.equals(output, encoded));
// Now let's try decode.
in = new ByteArrayInputStream(encoded);
in = new Base64InputStream(in);
output = new byte[decoded.length];
for (int i = 0; i < output.length; i++) {
output[i] = (byte) in.read();
}
assertEquals("EOF", -1, in.read());
assertEquals("Still EOF", -1, in.read());
assertTrue("Streaming base64 decode", Arrays.equals(output, decoded));
// I always wanted to do this! (wrap encoder with decoder etc etc).
in = new ByteArrayInputStream(decoded);
for (int i = 0; i < 10; i++) {
in = new Base64InputStream(in, true, chunkSize, seperator);
in = new Base64InputStream(in, false);
}
output = new byte[decoded.length];
for (int i = 0; i < output.length; i++) {
output[i] = (byte) in.read();
}
assertEquals("EOF", -1, in.read());
assertEquals("Still EOF", -1, in.read());
assertTrue("Streaming base64 wrap-wrap-wrap!", Arrays.equals(output, decoded));
}
/**
* Tests markSupported.
*
* @throws Exception
*/
public void testMarkSupported() throws Exception {
byte[] decoded = StringUtils.getBytesUtf8(STRING_FIXTURE);
ByteArrayInputStream bin = new ByteArrayInputStream(decoded);
Base64InputStream in = new Base64InputStream(bin, true, 4, new byte[]{0, 0, 0});
// Always returns false for now.
assertFalse("Base64InputStream.markSupported() is false", in.markSupported());
}
/**
* Tests read returning 0
*
* @throws Exception
*/
public void testRead0() throws Exception {
byte[] decoded = StringUtils.getBytesUtf8(STRING_FIXTURE);
byte[] buf = new byte[1024];
int bytesRead = 0;
ByteArrayInputStream bin = new ByteArrayInputStream(decoded);
Base64InputStream in = new Base64InputStream(bin, true, 4, new byte[]{0, 0, 0});
bytesRead = in.read(buf, 0, 0);
assertEquals("Base64InputStream.read(buf, 0, 0) returns 0", 0, bytesRead);
}
/**
* Tests read with null.
*
* @throws Exception
* for some failure scenarios.
*/
public void testReadNull() throws Exception {
byte[] decoded = StringUtils.getBytesUtf8(STRING_FIXTURE);
ByteArrayInputStream bin = new ByteArrayInputStream(decoded);
Base64InputStream in = new Base64InputStream(bin, true, 4, new byte[]{0, 0, 0});
try {
in.read(null, 0, 0);
fail("Base64InputStream.read(null, 0, 0) to throw a NullPointerException");
} catch (NullPointerException e) {
// Expected
}
}
/**
* Tests read throwing IndexOutOfBoundsException
*
* @throws Exception
*/
public void testReadOutOfBounds() throws Exception {
byte[] decoded = StringUtils.getBytesUtf8(STRING_FIXTURE);
byte[] buf = new byte[1024];
ByteArrayInputStream bin = new ByteArrayInputStream(decoded);
Base64InputStream in = new Base64InputStream(bin, true, 4, new byte[]{0, 0, 0});
try {
in.read(buf, -1, 0);
fail("Expected Base64InputStream.read(buf, -1, 0) to throw IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {
// Expected
}
try {
in.read(buf, 0, -1);
fail("Expected Base64InputStream.read(buf, 0, -1) to throw IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {
// Expected
}
try {
in.read(buf, buf.length + 1, 0);
fail("Base64InputStream.read(buf, buf.length + 1, 0) throws IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {
// Expected
}
try {
in.read(buf, buf.length - 1, 2);
fail("Base64InputStream.read(buf, buf.length - 1, 2) throws IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {
// Expected
}
}
} | // You are a professional Java test case writer, please create a test case named `testCodec98NPE` for the issue `Codec-CODEC-98`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Codec-CODEC-98
//
// ## Issue-Title:
// Base64InputStream causes NullPointerException on some input
//
// ## Issue-Description:
//
// Certain (malformed?) input to Base64InputStream causes a NullPointerException in Base64.decode.
//
//
// The exception occurs when Base64.decode is entered with the following conditions:
//
//
// * buffer is null
// * modulus is 3 from a previous entry.
// * inAvail is -1 because Base64InputStream.read reached EOF on line 150.
//
//
// Under these conditions, Base64.decode reaches line 581 with buffer still null and throws a NullPointerException.
//
//
// Here is some input data that will trigger it:
//
//
//
//
// ```
// H4sIAAAAAAAAAFvzloG1uIhBKiuxLFGvODW5tCizpFIvODM9LzXFPykrNbmE8//eDC2bq/+ZGJij
// GdiT8/NKUvNKShiYop2iGTiLgQoTS0qLUgsZ6hgYfRh4SjJSE3PS84GmZOSWMAj5gMzVz0nMS9cP
// LinKzEu3rigoLQJpXvNZ/AcbR8gDJgaGigIGBqbLayAuMUxNKdVLTyxJTc7QS07WSyzKLC7JL8lJ
// 1StJLErMKynNSdTLyUxOzStO1fOB0AwQwMjEwOrJwJMbn+mSWFkclpiTmeID4joml2SWpYZk5qaW
// MEj45Bel62flpyTqlwAF9F2A9oBkrMEqnYtSoXyob1hy4z1dShgEIL4oLcnM0Q8N9XQBqubKjYfa
// DjTV1AfoZn2Im/WTk/XhbtaHu1kf6mZ9T5g2YED8BwKgj8WAbtIDuUkP5CY9mJt22FSkZEXf/QkK
// oCIGeVRFSYlA/zsBCZjq//9/PvSP1VvMxMDkxcCe6ZuZk5NZ7MPAnemcUZSfl5+Tn15ZwiCF5n2E
// nDUoDhjVfhrpNABdpI5qWTJYmZ5nsD9Cg0pwSWnSyhOCaYXmAerMoDgsxnAkzG1R+XmpYPXL9Bln
// 1RhJPQarL+dgYNM1MLUyMKioKAYFOCvIBb8vl8qCOFxA4/jAiRIU7HqgYN8zk/n7jNxWfbAXeXJS
// E4tLgOnUKbOk2IuBOzcfzqso6M1QmrzKkedPzcYO3QZu129As4xITlZI6QqYFNhz44v9EkFpCGua
// LmEQdkktS83JL8gF5g4FqBGlIJ+wAI1gKJtZEvTws/j3FluPu4lcr7ra9OfHKXIZNTa4FPd8n33J
// QXPFLte9AZe5uBaJvGrKVl+rbrTaXDZO6NwU7gnHOVgzzsmnGX2Y5GDqrst8wcTear0Ab1yj6PrD
// F977vL/5iUMg773My5qLLK8OVAu6Tz7Xcyjy9Uym02Z/+xY7m85nYo/t4E93FXFKOf9/a3X78neS
// jE5Tu066K3Mdf17m66mbpXN9y34ZZ3ErRobfn+RfzVBIWj0vc82vY7YPvM5eLHHOulV77M6CoB4h
// xb/FjHWHRR+ldb6QmSP1ROGwGs+nx2quwitN7+mIps
public void testCodec98NPE() throws Exception {
| 66 | /**
* Test the Base64InputStream implementation against the special NPE inducing input
* identified in the CODEC-98 bug.
*
* @throws Exception for some failure scenarios.
*/ | 5 | 54 | src/test/org/apache/commons/codec/binary/Base64InputStreamTest.java | src/test | ```markdown
## Issue-ID: Codec-CODEC-98
## Issue-Title:
Base64InputStream causes NullPointerException on some input
## Issue-Description:
Certain (malformed?) input to Base64InputStream causes a NullPointerException in Base64.decode.
The exception occurs when Base64.decode is entered with the following conditions:
* buffer is null
* modulus is 3 from a previous entry.
* inAvail is -1 because Base64InputStream.read reached EOF on line 150.
Under these conditions, Base64.decode reaches line 581 with buffer still null and throws a NullPointerException.
Here is some input data that will trigger it:
```
H4sIAAAAAAAAAFvzloG1uIhBKiuxLFGvODW5tCizpFIvODM9LzXFPykrNbmE8//eDC2bq/+ZGJij
GdiT8/NKUvNKShiYop2iGTiLgQoTS0qLUgsZ6hgYfRh4SjJSE3PS84GmZOSWMAj5gMzVz0nMS9cP
LinKzEu3rigoLQJpXvNZ/AcbR8gDJgaGigIGBqbLayAuMUxNKdVLTyxJTc7QS07WSyzKLC7JL8lJ
1StJLErMKynNSdTLyUxOzStO1fOB0AwQwMjEwOrJwJMbn+mSWFkclpiTmeID4joml2SWpYZk5qaW
MEj45Bel62flpyTqlwAF9F2A9oBkrMEqnYtSoXyob1hy4z1dShgEIL4oLcnM0Q8N9XQBqubKjYfa
DjTV1AfoZn2Im/WTk/XhbtaHu1kf6mZ9T5g2YED8BwKgj8WAbtIDuUkP5CY9mJt22FSkZEXf/QkK
oCIGeVRFSYlA/zsBCZjq//9/PvSP1VvMxMDkxcCe6ZuZk5NZ7MPAnemcUZSfl5+Tn15ZwiCF5n2E
nDUoDhjVfhrpNABdpI5qWTJYmZ5nsD9Cg0pwSWnSyhOCaYXmAerMoDgsxnAkzG1R+XmpYPXL9Bln
1RhJPQarL+dgYNM1MLUyMKioKAYFOCvIBb8vl8qCOFxA4/jAiRIU7HqgYN8zk/n7jNxWfbAXeXJS
E4tLgOnUKbOk2IuBOzcfzqso6M1QmrzKkedPzcYO3QZu129As4xITlZI6QqYFNhz44v9EkFpCGua
LmEQdkktS83JL8gF5g4FqBGlIJ+wAI1gKJtZEvTws/j3FluPu4lcr7ra9OfHKXIZNTa4FPd8n33J
QXPFLte9AZe5uBaJvGrKVl+rbrTaXDZO6NwU7gnHOVgzzsmnGX2Y5GDqrst8wcTear0Ab1yj6PrD
F977vL/5iUMg773My5qLLK8OVAu6Tz7Xcyjy9Uym02Z/+xY7m85nYo/t4E93FXFKOf9/a3X78neS
jE5Tu066K3Mdf17m66mbpXN9y34ZZ3ErRobfn+RfzVBIWj0vc82vY7YPvM5eLHHOulV77M6CoB4h
xb/FjHWHRR+ldb6QmSP1ROGwGs+nx2quwitN7+mIps
```
You are a professional Java test case writer, please create a test case named `testCodec98NPE` for the issue `Codec-CODEC-98`, utilizing the provided issue report information and the following function signature.
```java
public void testCodec98NPE() throws Exception {
```
| 54 | [
"org.apache.commons.codec.binary.Base64"
] | 82d94101a7c7162487c62daf67c3fa04a448ce0d726867bfe2702a4493900a03 | public void testCodec98NPE() throws Exception | // You are a professional Java test case writer, please create a test case named `testCodec98NPE` for the issue `Codec-CODEC-98`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Codec-CODEC-98
//
// ## Issue-Title:
// Base64InputStream causes NullPointerException on some input
//
// ## Issue-Description:
//
// Certain (malformed?) input to Base64InputStream causes a NullPointerException in Base64.decode.
//
//
// The exception occurs when Base64.decode is entered with the following conditions:
//
//
// * buffer is null
// * modulus is 3 from a previous entry.
// * inAvail is -1 because Base64InputStream.read reached EOF on line 150.
//
//
// Under these conditions, Base64.decode reaches line 581 with buffer still null and throws a NullPointerException.
//
//
// Here is some input data that will trigger it:
//
//
//
//
// ```
// H4sIAAAAAAAAAFvzloG1uIhBKiuxLFGvODW5tCizpFIvODM9LzXFPykrNbmE8//eDC2bq/+ZGJij
// GdiT8/NKUvNKShiYop2iGTiLgQoTS0qLUgsZ6hgYfRh4SjJSE3PS84GmZOSWMAj5gMzVz0nMS9cP
// LinKzEu3rigoLQJpXvNZ/AcbR8gDJgaGigIGBqbLayAuMUxNKdVLTyxJTc7QS07WSyzKLC7JL8lJ
// 1StJLErMKynNSdTLyUxOzStO1fOB0AwQwMjEwOrJwJMbn+mSWFkclpiTmeID4joml2SWpYZk5qaW
// MEj45Bel62flpyTqlwAF9F2A9oBkrMEqnYtSoXyob1hy4z1dShgEIL4oLcnM0Q8N9XQBqubKjYfa
// DjTV1AfoZn2Im/WTk/XhbtaHu1kf6mZ9T5g2YED8BwKgj8WAbtIDuUkP5CY9mJt22FSkZEXf/QkK
// oCIGeVRFSYlA/zsBCZjq//9/PvSP1VvMxMDkxcCe6ZuZk5NZ7MPAnemcUZSfl5+Tn15ZwiCF5n2E
// nDUoDhjVfhrpNABdpI5qWTJYmZ5nsD9Cg0pwSWnSyhOCaYXmAerMoDgsxnAkzG1R+XmpYPXL9Bln
// 1RhJPQarL+dgYNM1MLUyMKioKAYFOCvIBb8vl8qCOFxA4/jAiRIU7HqgYN8zk/n7jNxWfbAXeXJS
// E4tLgOnUKbOk2IuBOzcfzqso6M1QmrzKkedPzcYO3QZu129As4xITlZI6QqYFNhz44v9EkFpCGua
// LmEQdkktS83JL8gF5g4FqBGlIJ+wAI1gKJtZEvTws/j3FluPu4lcr7ra9OfHKXIZNTa4FPd8n33J
// QXPFLte9AZe5uBaJvGrKVl+rbrTaXDZO6NwU7gnHOVgzzsmnGX2Y5GDqrst8wcTear0Ab1yj6PrD
// F977vL/5iUMg773My5qLLK8OVAu6Tz7Xcyjy9Uym02Z/+xY7m85nYo/t4E93FXFKOf9/a3X78neS
// jE5Tu066K3Mdf17m66mbpXN9y34ZZ3ErRobfn+RfzVBIWj0vc82vY7YPvM5eLHHOulV77M6CoB4h
// xb/FjHWHRR+ldb6QmSP1ROGwGs+nx2quwitN7+mIps
| Codec | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.codec.binary;
import java.io.ByteArrayInputStream;
import java.io.InputStream;
import java.util.Arrays;
import junit.framework.TestCase;
/**
* @author Apache Software Foundation
* @version $Id $
* @since 1.4
*/
public class Base64InputStreamTest extends TestCase {
private final static byte[] CRLF = {(byte) '\r', (byte) '\n'};
private final static byte[] LF = {(byte) '\n'};
private static final String STRING_FIXTURE = "Hello World";
/**
* Construct a new instance of this test case.
*
* @param name
* Name of the test case
*/
public Base64InputStreamTest(String name) {
super(name);
}
/**
* Test the Base64InputStream implementation against the special NPE inducing input
* identified in the CODEC-98 bug.
*
* @throws Exception for some failure scenarios.
*/
public void testCodec98NPE() throws Exception {
byte[] codec98 = StringUtils.getBytesUtf8(Base64TestData.CODEC_98_NPE);
ByteArrayInputStream data = new ByteArrayInputStream(codec98);
Base64InputStream stream = new Base64InputStream(data);
// This line causes an NPE in commons-codec-1.4.jar:
byte[] decodedBytes = Base64TestData.streamToBytes(stream, new byte[1024]);
String decoded = StringUtils.newStringUtf8(decodedBytes);
assertEquals(
"codec-98 NPE Base64InputStream", Base64TestData.CODEC_98_NPE_DECODED, decoded
);
}
/**
* Tests the Base64InputStream implementation against empty input.
*
* @throws Exception
* for some failure scenarios.
*/
public void testBase64EmptyInputStreamMimeChuckSize() throws Exception {
testBase64EmptyInputStream(Base64.MIME_CHUNK_SIZE);
}
/**
* Tests the Base64InputStream implementation against empty input.
*
* @throws Exception
* for some failure scenarios.
*/
public void testBase64EmptyInputStreamPemChuckSize() throws Exception {
testBase64EmptyInputStream(Base64.PEM_CHUNK_SIZE);
}
private void testBase64EmptyInputStream(int chuckSize) throws Exception {
byte[] emptyEncoded = new byte[0];
byte[] emptyDecoded = new byte[0];
testByteByByte(emptyEncoded, emptyDecoded, chuckSize, CRLF);
testByChunk(emptyEncoded, emptyDecoded, chuckSize, CRLF);
}
/**
* Tests the Base64InputStream implementation.
*
* @throws Exception
* for some failure scenarios.
*/
public void testBase64InputStreamByChunk() throws Exception {
// Hello World test.
byte[] encoded = StringUtils.getBytesUtf8("SGVsbG8gV29ybGQ=\r\n");
byte[] decoded = StringUtils.getBytesUtf8(STRING_FIXTURE);
testByChunk(encoded, decoded, Base64.MIME_CHUNK_SIZE, CRLF);
// Single Byte test.
encoded = StringUtils.getBytesUtf8("AA==\r\n");
decoded = new byte[]{(byte) 0};
testByChunk(encoded, decoded, Base64.MIME_CHUNK_SIZE, CRLF);
// OpenSSL interop test.
encoded = StringUtils.getBytesUtf8(Base64TestData.ENCODED_64_CHARS_PER_LINE);
decoded = Base64TestData.DECODED;
testByChunk(encoded, decoded, Base64.PEM_CHUNK_SIZE, LF);
// Single Line test.
String singleLine = Base64TestData.ENCODED_64_CHARS_PER_LINE.replaceAll("\n", "");
encoded = StringUtils.getBytesUtf8(singleLine);
decoded = Base64TestData.DECODED;
testByChunk(encoded, decoded, 0, LF);
// test random data of sizes 0 thru 150
for (int i = 0; i <= 150; i++) {
byte[][] randomData = Base64TestData.randomData(i, false);
encoded = randomData[1];
decoded = randomData[0];
testByChunk(encoded, decoded, 0, LF);
}
}
/**
* Tests the Base64InputStream implementation.
*
* @throws Exception
* for some failure scenarios.
*/
public void testBase64InputStreamByteByByte() throws Exception {
// Hello World test.
byte[] encoded = StringUtils.getBytesUtf8("SGVsbG8gV29ybGQ=\r\n");
byte[] decoded = StringUtils.getBytesUtf8(STRING_FIXTURE);
testByteByByte(encoded, decoded, Base64.MIME_CHUNK_SIZE, CRLF);
// Single Byte test.
encoded = StringUtils.getBytesUtf8("AA==\r\n");
decoded = new byte[]{(byte) 0};
testByteByByte(encoded, decoded, Base64.MIME_CHUNK_SIZE, CRLF);
// OpenSSL interop test.
encoded = StringUtils.getBytesUtf8(Base64TestData.ENCODED_64_CHARS_PER_LINE);
decoded = Base64TestData.DECODED;
testByteByByte(encoded, decoded, Base64.PEM_CHUNK_SIZE, LF);
// Single Line test.
String singleLine = Base64TestData.ENCODED_64_CHARS_PER_LINE.replaceAll("\n", "");
encoded = StringUtils.getBytesUtf8(singleLine);
decoded = Base64TestData.DECODED;
testByteByByte(encoded, decoded, 0, LF);
// test random data of sizes 0 thru 150
for (int i = 0; i <= 150; i++) {
byte[][] randomData = Base64TestData.randomData(i, false);
encoded = randomData[1];
decoded = randomData[0];
testByteByByte(encoded, decoded, 0, LF);
}
}
/**
* Tests method does three tests on the supplied data: 1. encoded ---[DECODE]--> decoded 2. decoded ---[ENCODE]-->
* encoded 3. decoded ---[WRAP-WRAP-WRAP-etc...] --> decoded
* <p/>
* By "[WRAP-WRAP-WRAP-etc...]" we mean situation where the Base64InputStream wraps itself in encode and decode mode
* over and over again.
*
* @param encoded
* base64 encoded data
* @param decoded
* the data from above, but decoded
* @param chunkSize
* chunk size (line-length) of the base64 encoded data.
* @param seperator
* Line separator in the base64 encoded data.
* @throws Exception
* Usually signifies a bug in the Base64 commons-codec implementation.
*/
private void testByChunk(byte[] encoded, byte[] decoded, int chunkSize, byte[] seperator) throws Exception {
// Start with encode.
InputStream in = new ByteArrayInputStream(decoded);
in = new Base64InputStream(in, true, chunkSize, seperator);
byte[] output = Base64TestData.streamToBytes(in);
assertEquals("EOF", -1, in.read());
assertEquals("Still EOF", -1, in.read());
assertTrue("Streaming base64 encode", Arrays.equals(output, encoded));
// Now let's try decode.
in = new ByteArrayInputStream(encoded);
in = new Base64InputStream(in);
output = Base64TestData.streamToBytes(in);
assertEquals("EOF", -1, in.read());
assertEquals("Still EOF", -1, in.read());
assertTrue("Streaming base64 decode", Arrays.equals(output, decoded));
// I always wanted to do this! (wrap encoder with decoder etc etc).
in = new ByteArrayInputStream(decoded);
for (int i = 0; i < 10; i++) {
in = new Base64InputStream(in, true, chunkSize, seperator);
in = new Base64InputStream(in, false);
}
output = Base64TestData.streamToBytes(in);
assertEquals("EOF", -1, in.read());
assertEquals("Still EOF", -1, in.read());
assertTrue("Streaming base64 wrap-wrap-wrap!", Arrays.equals(output, decoded));
}
/**
* Tests method does three tests on the supplied data: 1. encoded ---[DECODE]--> decoded 2. decoded ---[ENCODE]-->
* encoded 3. decoded ---[WRAP-WRAP-WRAP-etc...] --> decoded
* <p/>
* By "[WRAP-WRAP-WRAP-etc...]" we mean situation where the Base64InputStream wraps itself in encode and decode mode
* over and over again.
*
* @param encoded
* base64 encoded data
* @param decoded
* the data from above, but decoded
* @param chunkSize
* chunk size (line-length) of the base64 encoded data.
* @param seperator
* Line separator in the base64 encoded data.
* @throws Exception
* Usually signifies a bug in the Base64 commons-codec implementation.
*/
private void testByteByByte(byte[] encoded, byte[] decoded, int chunkSize, byte[] seperator) throws Exception {
// Start with encode.
InputStream in = new ByteArrayInputStream(decoded);
in = new Base64InputStream(in, true, chunkSize, seperator);
byte[] output = new byte[encoded.length];
for (int i = 0; i < output.length; i++) {
output[i] = (byte) in.read();
}
assertEquals("EOF", -1, in.read());
assertEquals("Still EOF", -1, in.read());
assertTrue("Streaming base64 encode", Arrays.equals(output, encoded));
// Now let's try decode.
in = new ByteArrayInputStream(encoded);
in = new Base64InputStream(in);
output = new byte[decoded.length];
for (int i = 0; i < output.length; i++) {
output[i] = (byte) in.read();
}
assertEquals("EOF", -1, in.read());
assertEquals("Still EOF", -1, in.read());
assertTrue("Streaming base64 decode", Arrays.equals(output, decoded));
// I always wanted to do this! (wrap encoder with decoder etc etc).
in = new ByteArrayInputStream(decoded);
for (int i = 0; i < 10; i++) {
in = new Base64InputStream(in, true, chunkSize, seperator);
in = new Base64InputStream(in, false);
}
output = new byte[decoded.length];
for (int i = 0; i < output.length; i++) {
output[i] = (byte) in.read();
}
assertEquals("EOF", -1, in.read());
assertEquals("Still EOF", -1, in.read());
assertTrue("Streaming base64 wrap-wrap-wrap!", Arrays.equals(output, decoded));
}
/**
* Tests markSupported.
*
* @throws Exception
*/
public void testMarkSupported() throws Exception {
byte[] decoded = StringUtils.getBytesUtf8(STRING_FIXTURE);
ByteArrayInputStream bin = new ByteArrayInputStream(decoded);
Base64InputStream in = new Base64InputStream(bin, true, 4, new byte[]{0, 0, 0});
// Always returns false for now.
assertFalse("Base64InputStream.markSupported() is false", in.markSupported());
}
/**
* Tests read returning 0
*
* @throws Exception
*/
public void testRead0() throws Exception {
byte[] decoded = StringUtils.getBytesUtf8(STRING_FIXTURE);
byte[] buf = new byte[1024];
int bytesRead = 0;
ByteArrayInputStream bin = new ByteArrayInputStream(decoded);
Base64InputStream in = new Base64InputStream(bin, true, 4, new byte[]{0, 0, 0});
bytesRead = in.read(buf, 0, 0);
assertEquals("Base64InputStream.read(buf, 0, 0) returns 0", 0, bytesRead);
}
/**
* Tests read with null.
*
* @throws Exception
* for some failure scenarios.
*/
public void testReadNull() throws Exception {
byte[] decoded = StringUtils.getBytesUtf8(STRING_FIXTURE);
ByteArrayInputStream bin = new ByteArrayInputStream(decoded);
Base64InputStream in = new Base64InputStream(bin, true, 4, new byte[]{0, 0, 0});
try {
in.read(null, 0, 0);
fail("Base64InputStream.read(null, 0, 0) to throw a NullPointerException");
} catch (NullPointerException e) {
// Expected
}
}
/**
* Tests read throwing IndexOutOfBoundsException
*
* @throws Exception
*/
public void testReadOutOfBounds() throws Exception {
byte[] decoded = StringUtils.getBytesUtf8(STRING_FIXTURE);
byte[] buf = new byte[1024];
ByteArrayInputStream bin = new ByteArrayInputStream(decoded);
Base64InputStream in = new Base64InputStream(bin, true, 4, new byte[]{0, 0, 0});
try {
in.read(buf, -1, 0);
fail("Expected Base64InputStream.read(buf, -1, 0) to throw IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {
// Expected
}
try {
in.read(buf, 0, -1);
fail("Expected Base64InputStream.read(buf, 0, -1) to throw IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {
// Expected
}
try {
in.read(buf, buf.length + 1, 0);
fail("Base64InputStream.read(buf, buf.length + 1, 0) throws IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {
// Expected
}
try {
in.read(buf, buf.length - 1, 2);
fail("Base64InputStream.read(buf, buf.length - 1, 2) throws IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {
// Expected
}
}
} |
|
@Test
public void testMath828Cycle() {
LinearObjectiveFunction f = new LinearObjectiveFunction(
new double[] { 1.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0}, 0.0);
ArrayList <LinearConstraint>constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] {0.0, 16.0, 14.0, 69.0, 1.0, 85.0, 52.0, 43.0, 64.0, 97.0, 14.0, 74.0, 89.0, 28.0, 94.0, 58.0, 13.0, 22.0, 21.0, 17.0, 30.0, 25.0, 1.0, 59.0, 91.0, 78.0, 12.0, 74.0, 56.0, 3.0, 88.0,}, Relationship.GEQ, 91.0));
constraints.add(new LinearConstraint(new double[] {0.0, 60.0, 40.0, 81.0, 71.0, 72.0, 46.0, 45.0, 38.0, 48.0, 40.0, 17.0, 33.0, 85.0, 64.0, 32.0, 84.0, 3.0, 54.0, 44.0, 71.0, 67.0, 90.0, 95.0, 54.0, 99.0, 99.0, 29.0, 52.0, 98.0, 9.0,}, Relationship.GEQ, 54.0));
constraints.add(new LinearConstraint(new double[] {0.0, 41.0, 12.0, 86.0, 90.0, 61.0, 31.0, 41.0, 23.0, 89.0, 17.0, 74.0, 44.0, 27.0, 16.0, 47.0, 80.0, 32.0, 11.0, 56.0, 68.0, 82.0, 11.0, 62.0, 62.0, 53.0, 39.0, 16.0, 48.0, 1.0, 63.0,}, Relationship.GEQ, 62.0));
constraints.add(new LinearConstraint(new double[] {83.0, -76.0, -94.0, -19.0, -15.0, -70.0, -72.0, -57.0, -63.0, -65.0, -22.0, -94.0, -22.0, -88.0, -86.0, -89.0, -72.0, -16.0, -80.0, -49.0, -70.0, -93.0, -95.0, -17.0, -83.0, -97.0, -31.0, -47.0, -31.0, -13.0, -23.0,}, Relationship.GEQ, 0.0));
constraints.add(new LinearConstraint(new double[] {41.0, -96.0, -41.0, -48.0, -70.0, -43.0, -43.0, -43.0, -97.0, -37.0, -85.0, -70.0, -45.0, -67.0, -87.0, -69.0, -94.0, -54.0, -54.0, -92.0, -79.0, -10.0, -35.0, -20.0, -41.0, -41.0, -65.0, -25.0, -12.0, -8.0, -46.0,}, Relationship.GEQ, 0.0));
constraints.add(new LinearConstraint(new double[] {27.0, -42.0, -65.0, -49.0, -53.0, -42.0, -17.0, -2.0, -61.0, -31.0, -76.0, -47.0, -8.0, -93.0, -86.0, -62.0, -65.0, -63.0, -22.0, -43.0, -27.0, -23.0, -32.0, -74.0, -27.0, -63.0, -47.0, -78.0, -29.0, -95.0, -73.0,}, Relationship.GEQ, 0.0));
constraints.add(new LinearConstraint(new double[] {15.0, -46.0, -41.0, -83.0, -98.0, -99.0, -21.0, -35.0, -7.0, -14.0, -80.0, -63.0, -18.0, -42.0, -5.0, -34.0, -56.0, -70.0, -16.0, -18.0, -74.0, -61.0, -47.0, -41.0, -15.0, -79.0, -18.0, -47.0, -88.0, -68.0, -55.0,}, Relationship.GEQ, 0.0));
double epsilon = 1e-6;
PointValuePair solution = new SimplexSolver().optimize(f, constraints, GoalType.MINIMIZE, true);
Assert.assertEquals(1.0d, solution.getValue(), epsilon);
Assert.assertTrue(validSolution(solution, constraints, epsilon));
} | org.apache.commons.math3.optimization.linear.SimplexSolverTest::testMath828Cycle | src/test/java/org/apache/commons/math3/optimization/linear/SimplexSolverTest.java | 72 | src/test/java/org/apache/commons/math3/optimization/linear/SimplexSolverTest.java | testMath828Cycle | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.math3.optimization.linear;
import org.junit.Assert;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import org.apache.commons.math3.optimization.GoalType;
import org.apache.commons.math3.optimization.PointValuePair;
import org.apache.commons.math3.util.Precision;
import org.junit.Test;
public class SimplexSolverTest {
@Test
public void testMath828() {
LinearObjectiveFunction f = new LinearObjectiveFunction(
new double[] { 1.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0}, 0.0);
ArrayList <LinearConstraint>constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] {0.0, 39.0, 23.0, 96.0, 15.0, 48.0, 9.0, 21.0, 48.0, 36.0, 76.0, 19.0, 88.0, 17.0, 16.0, 36.0,}, Relationship.GEQ, 15.0));
constraints.add(new LinearConstraint(new double[] {0.0, 59.0, 93.0, 12.0, 29.0, 78.0, 73.0, 87.0, 32.0, 70.0, 68.0, 24.0, 11.0, 26.0, 65.0, 25.0,}, Relationship.GEQ, 29.0));
constraints.add(new LinearConstraint(new double[] {0.0, 74.0, 5.0, 82.0, 6.0, 97.0, 55.0, 44.0, 52.0, 54.0, 5.0, 93.0, 91.0, 8.0, 20.0, 97.0,}, Relationship.GEQ, 6.0));
constraints.add(new LinearConstraint(new double[] {8.0, -3.0, -28.0, -72.0, -8.0, -31.0, -31.0, -74.0, -47.0, -59.0, -24.0, -57.0, -56.0, -16.0, -92.0, -59.0,}, Relationship.GEQ, 0.0));
constraints.add(new LinearConstraint(new double[] {25.0, -7.0, -99.0, -78.0, -25.0, -14.0, -16.0, -89.0, -39.0, -56.0, -53.0, -9.0, -18.0, -26.0, -11.0, -61.0,}, Relationship.GEQ, 0.0));
constraints.add(new LinearConstraint(new double[] {33.0, -95.0, -15.0, -4.0, -33.0, -3.0, -20.0, -96.0, -27.0, -13.0, -80.0, -24.0, -3.0, -13.0, -57.0, -76.0,}, Relationship.GEQ, 0.0));
constraints.add(new LinearConstraint(new double[] {7.0, -95.0, -39.0, -93.0, -7.0, -94.0, -94.0, -62.0, -76.0, -26.0, -53.0, -57.0, -31.0, -76.0, -53.0, -52.0,}, Relationship.GEQ, 0.0));
double epsilon = 1e-6;
PointValuePair solution = new SimplexSolver().optimize(f, constraints, GoalType.MINIMIZE, true);
Assert.assertEquals(1.0d, solution.getValue(), epsilon);
Assert.assertTrue(validSolution(solution, constraints, epsilon));
}
@Test
public void testMath828Cycle() {
LinearObjectiveFunction f = new LinearObjectiveFunction(
new double[] { 1.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0}, 0.0);
ArrayList <LinearConstraint>constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] {0.0, 16.0, 14.0, 69.0, 1.0, 85.0, 52.0, 43.0, 64.0, 97.0, 14.0, 74.0, 89.0, 28.0, 94.0, 58.0, 13.0, 22.0, 21.0, 17.0, 30.0, 25.0, 1.0, 59.0, 91.0, 78.0, 12.0, 74.0, 56.0, 3.0, 88.0,}, Relationship.GEQ, 91.0));
constraints.add(new LinearConstraint(new double[] {0.0, 60.0, 40.0, 81.0, 71.0, 72.0, 46.0, 45.0, 38.0, 48.0, 40.0, 17.0, 33.0, 85.0, 64.0, 32.0, 84.0, 3.0, 54.0, 44.0, 71.0, 67.0, 90.0, 95.0, 54.0, 99.0, 99.0, 29.0, 52.0, 98.0, 9.0,}, Relationship.GEQ, 54.0));
constraints.add(new LinearConstraint(new double[] {0.0, 41.0, 12.0, 86.0, 90.0, 61.0, 31.0, 41.0, 23.0, 89.0, 17.0, 74.0, 44.0, 27.0, 16.0, 47.0, 80.0, 32.0, 11.0, 56.0, 68.0, 82.0, 11.0, 62.0, 62.0, 53.0, 39.0, 16.0, 48.0, 1.0, 63.0,}, Relationship.GEQ, 62.0));
constraints.add(new LinearConstraint(new double[] {83.0, -76.0, -94.0, -19.0, -15.0, -70.0, -72.0, -57.0, -63.0, -65.0, -22.0, -94.0, -22.0, -88.0, -86.0, -89.0, -72.0, -16.0, -80.0, -49.0, -70.0, -93.0, -95.0, -17.0, -83.0, -97.0, -31.0, -47.0, -31.0, -13.0, -23.0,}, Relationship.GEQ, 0.0));
constraints.add(new LinearConstraint(new double[] {41.0, -96.0, -41.0, -48.0, -70.0, -43.0, -43.0, -43.0, -97.0, -37.0, -85.0, -70.0, -45.0, -67.0, -87.0, -69.0, -94.0, -54.0, -54.0, -92.0, -79.0, -10.0, -35.0, -20.0, -41.0, -41.0, -65.0, -25.0, -12.0, -8.0, -46.0,}, Relationship.GEQ, 0.0));
constraints.add(new LinearConstraint(new double[] {27.0, -42.0, -65.0, -49.0, -53.0, -42.0, -17.0, -2.0, -61.0, -31.0, -76.0, -47.0, -8.0, -93.0, -86.0, -62.0, -65.0, -63.0, -22.0, -43.0, -27.0, -23.0, -32.0, -74.0, -27.0, -63.0, -47.0, -78.0, -29.0, -95.0, -73.0,}, Relationship.GEQ, 0.0));
constraints.add(new LinearConstraint(new double[] {15.0, -46.0, -41.0, -83.0, -98.0, -99.0, -21.0, -35.0, -7.0, -14.0, -80.0, -63.0, -18.0, -42.0, -5.0, -34.0, -56.0, -70.0, -16.0, -18.0, -74.0, -61.0, -47.0, -41.0, -15.0, -79.0, -18.0, -47.0, -88.0, -68.0, -55.0,}, Relationship.GEQ, 0.0));
double epsilon = 1e-6;
PointValuePair solution = new SimplexSolver().optimize(f, constraints, GoalType.MINIMIZE, true);
Assert.assertEquals(1.0d, solution.getValue(), epsilon);
Assert.assertTrue(validSolution(solution, constraints, epsilon));
}
@Test
public void testMath781() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 2, 6, 7 }, 0);
ArrayList<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 2, 1 }, Relationship.LEQ, 2));
constraints.add(new LinearConstraint(new double[] { -1, 1, 1 }, Relationship.LEQ, -1));
constraints.add(new LinearConstraint(new double[] { 2, -3, 1 }, Relationship.LEQ, -1));
double epsilon = 1e-6;
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, false);
Assert.assertTrue(Precision.compareTo(solution.getPoint()[0], 0.0d, epsilon) > 0);
Assert.assertTrue(Precision.compareTo(solution.getPoint()[1], 0.0d, epsilon) > 0);
Assert.assertTrue(Precision.compareTo(solution.getPoint()[2], 0.0d, epsilon) < 0);
Assert.assertEquals(2.0d, solution.getValue(), epsilon);
}
@Test
public void testMath713NegativeVariable() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] {1.0, 1.0}, 0.0d);
ArrayList<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] {1, 0}, Relationship.EQ, 1));
double epsilon = 1e-6;
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MINIMIZE, true);
Assert.assertTrue(Precision.compareTo(solution.getPoint()[0], 0.0d, epsilon) >= 0);
Assert.assertTrue(Precision.compareTo(solution.getPoint()[1], 0.0d, epsilon) >= 0);
}
@Test
public void testMath434NegativeVariable() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] {0.0, 0.0, 1.0}, 0.0d);
ArrayList<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] {1, 1, 0}, Relationship.EQ, 5));
constraints.add(new LinearConstraint(new double[] {0, 0, 1}, Relationship.GEQ, -10));
double epsilon = 1e-6;
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MINIMIZE, false);
Assert.assertEquals(5.0, solution.getPoint()[0] + solution.getPoint()[1], epsilon);
Assert.assertEquals(-10.0, solution.getPoint()[2], epsilon);
Assert.assertEquals(-10.0, solution.getValue(), epsilon);
}
@Test(expected = NoFeasibleSolutionException.class)
public void testMath434UnfeasibleSolution() {
double epsilon = 1e-6;
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] {1.0, 0.0}, 0.0);
ArrayList<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] {epsilon/2, 0.5}, Relationship.EQ, 0));
constraints.add(new LinearConstraint(new double[] {1e-3, 0.1}, Relationship.EQ, 10));
SimplexSolver solver = new SimplexSolver();
// allowing only non-negative values, no feasible solution shall be found
solver.optimize(f, constraints, GoalType.MINIMIZE, true);
}
@Test
public void testMath434PivotRowSelection() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] {1.0}, 0.0);
double epsilon = 1e-6;
ArrayList<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] {200}, Relationship.GEQ, 1));
constraints.add(new LinearConstraint(new double[] {100}, Relationship.GEQ, 0.499900001));
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MINIMIZE, false);
Assert.assertTrue(Precision.compareTo(solution.getPoint()[0] * 200.d, 1.d, epsilon) >= 0);
Assert.assertEquals(0.0050, solution.getValue(), epsilon);
}
@Test
public void testMath434PivotRowSelection2() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] {0.0d, 1.0d, 1.0d, 0.0d, 0.0d, 0.0d, 0.0d}, 0.0d);
ArrayList<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] {1.0d, -0.1d, 0.0d, 0.0d, 0.0d, 0.0d, 0.0d}, Relationship.EQ, -0.1d));
constraints.add(new LinearConstraint(new double[] {1.0d, 0.0d, 0.0d, 0.0d, 0.0d, 0.0d, 0.0d}, Relationship.GEQ, -1e-18d));
constraints.add(new LinearConstraint(new double[] {0.0d, 1.0d, 0.0d, 0.0d, 0.0d, 0.0d, 0.0d}, Relationship.GEQ, 0.0d));
constraints.add(new LinearConstraint(new double[] {0.0d, 0.0d, 0.0d, 1.0d, 0.0d, -0.0128588d, 1e-5d}, Relationship.EQ, 0.0d));
constraints.add(new LinearConstraint(new double[] {0.0d, 0.0d, 0.0d, 0.0d, 1.0d, 1e-5d, -0.0128586d}, Relationship.EQ, 1e-10d));
constraints.add(new LinearConstraint(new double[] {0.0d, 0.0d, 1.0d, -1.0d, 0.0d, 0.0d, 0.0d}, Relationship.GEQ, 0.0d));
constraints.add(new LinearConstraint(new double[] {0.0d, 0.0d, 1.0d, 1.0d, 0.0d, 0.0d, 0.0d}, Relationship.GEQ, 0.0d));
constraints.add(new LinearConstraint(new double[] {0.0d, 0.0d, 1.0d, 0.0d, -1.0d, 0.0d, 0.0d}, Relationship.GEQ, 0.0d));
constraints.add(new LinearConstraint(new double[] {0.0d, 0.0d, 1.0d, 0.0d, 1.0d, 0.0d, 0.0d}, Relationship.GEQ, 0.0d));
double epsilon = 1e-7;
SimplexSolver simplex = new SimplexSolver();
PointValuePair solution = simplex.optimize(f, constraints, GoalType.MINIMIZE, false);
Assert.assertTrue(Precision.compareTo(solution.getPoint()[0], -1e-18d, epsilon) >= 0);
Assert.assertEquals(1.0d, solution.getPoint()[1], epsilon);
Assert.assertEquals(0.0d, solution.getPoint()[2], epsilon);
Assert.assertEquals(1.0d, solution.getValue(), epsilon);
}
@Test
public void testMath272() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 2, 2, 1 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 1, 0 }, Relationship.GEQ, 1));
constraints.add(new LinearConstraint(new double[] { 1, 0, 1 }, Relationship.GEQ, 1));
constraints.add(new LinearConstraint(new double[] { 0, 1, 0 }, Relationship.GEQ, 1));
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MINIMIZE, true);
Assert.assertEquals(0.0, solution.getPoint()[0], .0000001);
Assert.assertEquals(1.0, solution.getPoint()[1], .0000001);
Assert.assertEquals(1.0, solution.getPoint()[2], .0000001);
Assert.assertEquals(3.0, solution.getValue(), .0000001);
}
@Test
public void testMath286() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 0.8, 0.2, 0.7, 0.3, 0.6, 0.4 }, 0 );
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 0, 1, 0, 1, 0 }, Relationship.EQ, 23.0));
constraints.add(new LinearConstraint(new double[] { 0, 1, 0, 1, 0, 1 }, Relationship.EQ, 23.0));
constraints.add(new LinearConstraint(new double[] { 1, 0, 0, 0, 0, 0 }, Relationship.GEQ, 10.0));
constraints.add(new LinearConstraint(new double[] { 0, 0, 1, 0, 0, 0 }, Relationship.GEQ, 8.0));
constraints.add(new LinearConstraint(new double[] { 0, 0, 0, 0, 1, 0 }, Relationship.GEQ, 5.0));
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, true);
Assert.assertEquals(25.8, solution.getValue(), .0000001);
Assert.assertEquals(23.0, solution.getPoint()[0] + solution.getPoint()[2] + solution.getPoint()[4], 0.0000001);
Assert.assertEquals(23.0, solution.getPoint()[1] + solution.getPoint()[3] + solution.getPoint()[5], 0.0000001);
Assert.assertTrue(solution.getPoint()[0] >= 10.0 - 0.0000001);
Assert.assertTrue(solution.getPoint()[2] >= 8.0 - 0.0000001);
Assert.assertTrue(solution.getPoint()[4] >= 5.0 - 0.0000001);
}
@Test
public void testDegeneracy() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 0.8, 0.7 }, 0 );
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 1 }, Relationship.LEQ, 18.0));
constraints.add(new LinearConstraint(new double[] { 1, 0 }, Relationship.GEQ, 10.0));
constraints.add(new LinearConstraint(new double[] { 0, 1 }, Relationship.GEQ, 8.0));
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, true);
Assert.assertEquals(13.6, solution.getValue(), .0000001);
}
@Test
public void testMath288() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 7, 3, 0, 0 }, 0 );
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 3, 0, -5, 0 }, Relationship.LEQ, 0.0));
constraints.add(new LinearConstraint(new double[] { 2, 0, 0, -5 }, Relationship.LEQ, 0.0));
constraints.add(new LinearConstraint(new double[] { 0, 3, 0, -5 }, Relationship.LEQ, 0.0));
constraints.add(new LinearConstraint(new double[] { 1, 0, 0, 0 }, Relationship.LEQ, 1.0));
constraints.add(new LinearConstraint(new double[] { 0, 1, 0, 0 }, Relationship.LEQ, 1.0));
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, true);
Assert.assertEquals(10.0, solution.getValue(), .0000001);
}
@Test
public void testMath290GEQ() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 1, 5 }, 0 );
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 2, 0 }, Relationship.GEQ, -1.0));
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MINIMIZE, true);
Assert.assertEquals(0, solution.getValue(), .0000001);
Assert.assertEquals(0, solution.getPoint()[0], .0000001);
Assert.assertEquals(0, solution.getPoint()[1], .0000001);
}
@Test(expected=NoFeasibleSolutionException.class)
public void testMath290LEQ() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 1, 5 }, 0 );
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 2, 0 }, Relationship.LEQ, -1.0));
SimplexSolver solver = new SimplexSolver();
solver.optimize(f, constraints, GoalType.MINIMIZE, true);
}
@Test
public void testMath293() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 0.8, 0.2, 0.7, 0.3, 0.4, 0.6}, 0 );
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 0, 1, 0, 1, 0 }, Relationship.EQ, 30.0));
constraints.add(new LinearConstraint(new double[] { 0, 1, 0, 1, 0, 1 }, Relationship.EQ, 30.0));
constraints.add(new LinearConstraint(new double[] { 0.8, 0.2, 0.0, 0.0, 0.0, 0.0 }, Relationship.GEQ, 10.0));
constraints.add(new LinearConstraint(new double[] { 0.0, 0.0, 0.7, 0.3, 0.0, 0.0 }, Relationship.GEQ, 10.0));
constraints.add(new LinearConstraint(new double[] { 0.0, 0.0, 0.0, 0.0, 0.4, 0.6 }, Relationship.GEQ, 10.0));
SimplexSolver solver = new SimplexSolver();
PointValuePair solution1 = solver.optimize(f, constraints, GoalType.MAXIMIZE, true);
Assert.assertEquals(15.7143, solution1.getPoint()[0], .0001);
Assert.assertEquals(0.0, solution1.getPoint()[1], .0001);
Assert.assertEquals(14.2857, solution1.getPoint()[2], .0001);
Assert.assertEquals(0.0, solution1.getPoint()[3], .0001);
Assert.assertEquals(0.0, solution1.getPoint()[4], .0001);
Assert.assertEquals(30.0, solution1.getPoint()[5], .0001);
Assert.assertEquals(40.57143, solution1.getValue(), .0001);
double valA = 0.8 * solution1.getPoint()[0] + 0.2 * solution1.getPoint()[1];
double valB = 0.7 * solution1.getPoint()[2] + 0.3 * solution1.getPoint()[3];
double valC = 0.4 * solution1.getPoint()[4] + 0.6 * solution1.getPoint()[5];
f = new LinearObjectiveFunction(new double[] { 0.8, 0.2, 0.7, 0.3, 0.4, 0.6}, 0 );
constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 0, 1, 0, 1, 0 }, Relationship.EQ, 30.0));
constraints.add(new LinearConstraint(new double[] { 0, 1, 0, 1, 0, 1 }, Relationship.EQ, 30.0));
constraints.add(new LinearConstraint(new double[] { 0.8, 0.2, 0.0, 0.0, 0.0, 0.0 }, Relationship.GEQ, valA));
constraints.add(new LinearConstraint(new double[] { 0.0, 0.0, 0.7, 0.3, 0.0, 0.0 }, Relationship.GEQ, valB));
constraints.add(new LinearConstraint(new double[] { 0.0, 0.0, 0.0, 0.0, 0.4, 0.6 }, Relationship.GEQ, valC));
PointValuePair solution2 = solver.optimize(f, constraints, GoalType.MAXIMIZE, true);
Assert.assertEquals(40.57143, solution2.getValue(), .0001);
}
@Test
public void testSimplexSolver() {
LinearObjectiveFunction f =
new LinearObjectiveFunction(new double[] { 15, 10 }, 7);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 0 }, Relationship.LEQ, 2));
constraints.add(new LinearConstraint(new double[] { 0, 1 }, Relationship.LEQ, 3));
constraints.add(new LinearConstraint(new double[] { 1, 1 }, Relationship.EQ, 4));
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, false);
Assert.assertEquals(2.0, solution.getPoint()[0], 0.0);
Assert.assertEquals(2.0, solution.getPoint()[1], 0.0);
Assert.assertEquals(57.0, solution.getValue(), 0.0);
}
@Test
public void testSingleVariableAndConstraint() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 3 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1 }, Relationship.LEQ, 10));
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, false);
Assert.assertEquals(10.0, solution.getPoint()[0], 0.0);
Assert.assertEquals(30.0, solution.getValue(), 0.0);
}
/**
* With no artificial variables needed (no equals and no greater than
* constraints) we can go straight to Phase 2.
*/
@Test
public void testModelWithNoArtificialVars() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 15, 10 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 0 }, Relationship.LEQ, 2));
constraints.add(new LinearConstraint(new double[] { 0, 1 }, Relationship.LEQ, 3));
constraints.add(new LinearConstraint(new double[] { 1, 1 }, Relationship.LEQ, 4));
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, false);
Assert.assertEquals(2.0, solution.getPoint()[0], 0.0);
Assert.assertEquals(2.0, solution.getPoint()[1], 0.0);
Assert.assertEquals(50.0, solution.getValue(), 0.0);
}
@Test
public void testMinimization() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { -2, 1 }, -5);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 2 }, Relationship.LEQ, 6));
constraints.add(new LinearConstraint(new double[] { 3, 2 }, Relationship.LEQ, 12));
constraints.add(new LinearConstraint(new double[] { 0, 1 }, Relationship.GEQ, 0));
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MINIMIZE, false);
Assert.assertEquals(4.0, solution.getPoint()[0], 0.0);
Assert.assertEquals(0.0, solution.getPoint()[1], 0.0);
Assert.assertEquals(-13.0, solution.getValue(), 0.0);
}
@Test
public void testSolutionWithNegativeDecisionVariable() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { -2, 1 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 1 }, Relationship.GEQ, 6));
constraints.add(new LinearConstraint(new double[] { 1, 2 }, Relationship.LEQ, 14));
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, false);
Assert.assertEquals(-2.0, solution.getPoint()[0], 0.0);
Assert.assertEquals(8.0, solution.getPoint()[1], 0.0);
Assert.assertEquals(12.0, solution.getValue(), 0.0);
}
@Test(expected = NoFeasibleSolutionException.class)
public void testInfeasibleSolution() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 15 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1 }, Relationship.LEQ, 1));
constraints.add(new LinearConstraint(new double[] { 1 }, Relationship.GEQ, 3));
SimplexSolver solver = new SimplexSolver();
solver.optimize(f, constraints, GoalType.MAXIMIZE, false);
}
@Test(expected = UnboundedSolutionException.class)
public void testUnboundedSolution() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 15, 10 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 0 }, Relationship.EQ, 2));
SimplexSolver solver = new SimplexSolver();
solver.optimize(f, constraints, GoalType.MAXIMIZE, false);
}
@Test
public void testRestrictVariablesToNonNegative() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 409, 523, 70, 204, 339 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 43, 56, 345, 56, 5 }, Relationship.LEQ, 4567456));
constraints.add(new LinearConstraint(new double[] { 12, 45, 7, 56, 23 }, Relationship.LEQ, 56454));
constraints.add(new LinearConstraint(new double[] { 8, 768, 0, 34, 7456 }, Relationship.LEQ, 1923421));
constraints.add(new LinearConstraint(new double[] { 12342, 2342, 34, 678, 2342 }, Relationship.GEQ, 4356));
constraints.add(new LinearConstraint(new double[] { 45, 678, 76, 52, 23 }, Relationship.EQ, 456356));
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, true);
Assert.assertEquals(2902.92783505155, solution.getPoint()[0], .0000001);
Assert.assertEquals(480.419243986254, solution.getPoint()[1], .0000001);
Assert.assertEquals(0.0, solution.getPoint()[2], .0000001);
Assert.assertEquals(0.0, solution.getPoint()[3], .0000001);
Assert.assertEquals(0.0, solution.getPoint()[4], .0000001);
Assert.assertEquals(1438556.7491409, solution.getValue(), .0000001);
}
@Test
public void testEpsilon() {
LinearObjectiveFunction f =
new LinearObjectiveFunction(new double[] { 10, 5, 1 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 9, 8, 0 }, Relationship.EQ, 17));
constraints.add(new LinearConstraint(new double[] { 0, 7, 8 }, Relationship.LEQ, 7));
constraints.add(new LinearConstraint(new double[] { 10, 0, 2 }, Relationship.LEQ, 10));
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, false);
Assert.assertEquals(1.0, solution.getPoint()[0], 0.0);
Assert.assertEquals(1.0, solution.getPoint()[1], 0.0);
Assert.assertEquals(0.0, solution.getPoint()[2], 0.0);
Assert.assertEquals(15.0, solution.getValue(), 0.0);
}
@Test
public void testTrivialModel() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 1, 1 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 1 }, Relationship.EQ, 0));
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, true);
Assert.assertEquals(0, solution.getValue(), .0000001);
}
@Test
public void testLargeModel() {
double[] objective = new double[] {
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 12, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
12, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 12, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 12, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 12, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 12, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1};
LinearObjectiveFunction f = new LinearObjectiveFunction(objective, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(equationFromString(objective.length, "x0 + x1 + x2 + x3 - x12 = 0"));
constraints.add(equationFromString(objective.length, "x4 + x5 + x6 + x7 + x8 + x9 + x10 + x11 - x13 = 0"));
constraints.add(equationFromString(objective.length, "x4 + x5 + x6 + x7 + x8 + x9 + x10 + x11 >= 49"));
constraints.add(equationFromString(objective.length, "x0 + x1 + x2 + x3 >= 42"));
constraints.add(equationFromString(objective.length, "x14 + x15 + x16 + x17 - x26 = 0"));
constraints.add(equationFromString(objective.length, "x18 + x19 + x20 + x21 + x22 + x23 + x24 + x25 - x27 = 0"));
constraints.add(equationFromString(objective.length, "x14 + x15 + x16 + x17 - x12 = 0"));
constraints.add(equationFromString(objective.length, "x18 + x19 + x20 + x21 + x22 + x23 + x24 + x25 - x13 = 0"));
constraints.add(equationFromString(objective.length, "x28 + x29 + x30 + x31 - x40 = 0"));
constraints.add(equationFromString(objective.length, "x32 + x33 + x34 + x35 + x36 + x37 + x38 + x39 - x41 = 0"));
constraints.add(equationFromString(objective.length, "x32 + x33 + x34 + x35 + x36 + x37 + x38 + x39 >= 49"));
constraints.add(equationFromString(objective.length, "x28 + x29 + x30 + x31 >= 42"));
constraints.add(equationFromString(objective.length, "x42 + x43 + x44 + x45 - x54 = 0"));
constraints.add(equationFromString(objective.length, "x46 + x47 + x48 + x49 + x50 + x51 + x52 + x53 - x55 = 0"));
constraints.add(equationFromString(objective.length, "x42 + x43 + x44 + x45 - x40 = 0"));
constraints.add(equationFromString(objective.length, "x46 + x47 + x48 + x49 + x50 + x51 + x52 + x53 - x41 = 0"));
constraints.add(equationFromString(objective.length, "x56 + x57 + x58 + x59 - x68 = 0"));
constraints.add(equationFromString(objective.length, "x60 + x61 + x62 + x63 + x64 + x65 + x66 + x67 - x69 = 0"));
constraints.add(equationFromString(objective.length, "x60 + x61 + x62 + x63 + x64 + x65 + x66 + x67 >= 51"));
constraints.add(equationFromString(objective.length, "x56 + x57 + x58 + x59 >= 44"));
constraints.add(equationFromString(objective.length, "x70 + x71 + x72 + x73 - x82 = 0"));
constraints.add(equationFromString(objective.length, "x74 + x75 + x76 + x77 + x78 + x79 + x80 + x81 - x83 = 0"));
constraints.add(equationFromString(objective.length, "x70 + x71 + x72 + x73 - x68 = 0"));
constraints.add(equationFromString(objective.length, "x74 + x75 + x76 + x77 + x78 + x79 + x80 + x81 - x69 = 0"));
constraints.add(equationFromString(objective.length, "x84 + x85 + x86 + x87 - x96 = 0"));
constraints.add(equationFromString(objective.length, "x88 + x89 + x90 + x91 + x92 + x93 + x94 + x95 - x97 = 0"));
constraints.add(equationFromString(objective.length, "x88 + x89 + x90 + x91 + x92 + x93 + x94 + x95 >= 51"));
constraints.add(equationFromString(objective.length, "x84 + x85 + x86 + x87 >= 44"));
constraints.add(equationFromString(objective.length, "x98 + x99 + x100 + x101 - x110 = 0"));
constraints.add(equationFromString(objective.length, "x102 + x103 + x104 + x105 + x106 + x107 + x108 + x109 - x111 = 0"));
constraints.add(equationFromString(objective.length, "x98 + x99 + x100 + x101 - x96 = 0"));
constraints.add(equationFromString(objective.length, "x102 + x103 + x104 + x105 + x106 + x107 + x108 + x109 - x97 = 0"));
constraints.add(equationFromString(objective.length, "x112 + x113 + x114 + x115 - x124 = 0"));
constraints.add(equationFromString(objective.length, "x116 + x117 + x118 + x119 + x120 + x121 + x122 + x123 - x125 = 0"));
constraints.add(equationFromString(objective.length, "x116 + x117 + x118 + x119 + x120 + x121 + x122 + x123 >= 49"));
constraints.add(equationFromString(objective.length, "x112 + x113 + x114 + x115 >= 42"));
constraints.add(equationFromString(objective.length, "x126 + x127 + x128 + x129 - x138 = 0"));
constraints.add(equationFromString(objective.length, "x130 + x131 + x132 + x133 + x134 + x135 + x136 + x137 - x139 = 0"));
constraints.add(equationFromString(objective.length, "x126 + x127 + x128 + x129 - x124 = 0"));
constraints.add(equationFromString(objective.length, "x130 + x131 + x132 + x133 + x134 + x135 + x136 + x137 - x125 = 0"));
constraints.add(equationFromString(objective.length, "x140 + x141 + x142 + x143 - x152 = 0"));
constraints.add(equationFromString(objective.length, "x144 + x145 + x146 + x147 + x148 + x149 + x150 + x151 - x153 = 0"));
constraints.add(equationFromString(objective.length, "x144 + x145 + x146 + x147 + x148 + x149 + x150 + x151 >= 59"));
constraints.add(equationFromString(objective.length, "x140 + x141 + x142 + x143 >= 42"));
constraints.add(equationFromString(objective.length, "x154 + x155 + x156 + x157 - x166 = 0"));
constraints.add(equationFromString(objective.length, "x158 + x159 + x160 + x161 + x162 + x163 + x164 + x165 - x167 = 0"));
constraints.add(equationFromString(objective.length, "x154 + x155 + x156 + x157 - x152 = 0"));
constraints.add(equationFromString(objective.length, "x158 + x159 + x160 + x161 + x162 + x163 + x164 + x165 - x153 = 0"));
constraints.add(equationFromString(objective.length, "x83 + x82 - x168 = 0"));
constraints.add(equationFromString(objective.length, "x111 + x110 - x169 = 0"));
constraints.add(equationFromString(objective.length, "x170 - x182 = 0"));
constraints.add(equationFromString(objective.length, "x171 - x183 = 0"));
constraints.add(equationFromString(objective.length, "x172 - x184 = 0"));
constraints.add(equationFromString(objective.length, "x173 - x185 = 0"));
constraints.add(equationFromString(objective.length, "x174 - x186 = 0"));
constraints.add(equationFromString(objective.length, "x175 + x176 - x187 = 0"));
constraints.add(equationFromString(objective.length, "x177 - x188 = 0"));
constraints.add(equationFromString(objective.length, "x178 - x189 = 0"));
constraints.add(equationFromString(objective.length, "x179 - x190 = 0"));
constraints.add(equationFromString(objective.length, "x180 - x191 = 0"));
constraints.add(equationFromString(objective.length, "x181 - x192 = 0"));
constraints.add(equationFromString(objective.length, "x170 - x26 = 0"));
constraints.add(equationFromString(objective.length, "x171 - x27 = 0"));
constraints.add(equationFromString(objective.length, "x172 - x54 = 0"));
constraints.add(equationFromString(objective.length, "x173 - x55 = 0"));
constraints.add(equationFromString(objective.length, "x174 - x168 = 0"));
constraints.add(equationFromString(objective.length, "x177 - x169 = 0"));
constraints.add(equationFromString(objective.length, "x178 - x138 = 0"));
constraints.add(equationFromString(objective.length, "x179 - x139 = 0"));
constraints.add(equationFromString(objective.length, "x180 - x166 = 0"));
constraints.add(equationFromString(objective.length, "x181 - x167 = 0"));
constraints.add(equationFromString(objective.length, "x193 - x205 = 0"));
constraints.add(equationFromString(objective.length, "x194 - x206 = 0"));
constraints.add(equationFromString(objective.length, "x195 - x207 = 0"));
constraints.add(equationFromString(objective.length, "x196 - x208 = 0"));
constraints.add(equationFromString(objective.length, "x197 - x209 = 0"));
constraints.add(equationFromString(objective.length, "x198 + x199 - x210 = 0"));
constraints.add(equationFromString(objective.length, "x200 - x211 = 0"));
constraints.add(equationFromString(objective.length, "x201 - x212 = 0"));
constraints.add(equationFromString(objective.length, "x202 - x213 = 0"));
constraints.add(equationFromString(objective.length, "x203 - x214 = 0"));
constraints.add(equationFromString(objective.length, "x204 - x215 = 0"));
constraints.add(equationFromString(objective.length, "x193 - x182 = 0"));
constraints.add(equationFromString(objective.length, "x194 - x183 = 0"));
constraints.add(equationFromString(objective.length, "x195 - x184 = 0"));
constraints.add(equationFromString(objective.length, "x196 - x185 = 0"));
constraints.add(equationFromString(objective.length, "x197 - x186 = 0"));
constraints.add(equationFromString(objective.length, "x198 + x199 - x187 = 0"));
constraints.add(equationFromString(objective.length, "x200 - x188 = 0"));
constraints.add(equationFromString(objective.length, "x201 - x189 = 0"));
constraints.add(equationFromString(objective.length, "x202 - x190 = 0"));
constraints.add(equationFromString(objective.length, "x203 - x191 = 0"));
constraints.add(equationFromString(objective.length, "x204 - x192 = 0"));
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MINIMIZE, true);
Assert.assertEquals(7518.0, solution.getValue(), .0000001);
}
/**
* Converts a test string to a {@link LinearConstraint}.
* Ex: x0 + x1 + x2 + x3 - x12 = 0
*/
private LinearConstraint equationFromString(int numCoefficients, String s) {
Relationship relationship;
if (s.contains(">=")) {
relationship = Relationship.GEQ;
} else if (s.contains("<=")) {
relationship = Relationship.LEQ;
} else if (s.contains("=")) {
relationship = Relationship.EQ;
} else {
throw new IllegalArgumentException();
}
String[] equationParts = s.split("[>|<]?=");
double rhs = Double.parseDouble(equationParts[1].trim());
double[] lhs = new double[numCoefficients];
String left = equationParts[0].replaceAll(" ?x", "");
String[] coefficients = left.split(" ");
for (String coefficient : coefficients) {
double value = coefficient.charAt(0) == '-' ? -1 : 1;
int index = Integer.parseInt(coefficient.replaceFirst("[+|-]", "").trim());
lhs[index] = value;
}
return new LinearConstraint(lhs, relationship, rhs);
}
private static boolean validSolution(PointValuePair solution, List<LinearConstraint> constraints, double epsilon) {
double[] vals = solution.getPoint();
for (LinearConstraint c : constraints) {
double[] coeffs = c.getCoefficients().toArray();
double result = 0.0d;
for (int i = 0; i < vals.length; i++) {
result += vals[i] * coeffs[i];
}
switch (c.getRelationship()) {
case EQ:
if (!Precision.equals(result, c.getValue(), epsilon)) {
return false;
}
break;
case GEQ:
if (Precision.compareTo(result, c.getValue(), epsilon) < 0) {
return false;
}
break;
case LEQ:
if (Precision.compareTo(result, c.getValue(), epsilon) > 0) {
return false;
}
break;
}
}
return true;
}
} | // You are a professional Java test case writer, please create a test case named `testMath828Cycle` for the issue `Math-MATH-828`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Math-MATH-828
//
// ## Issue-Title:
// Not expected UnboundedSolutionException
//
// ## Issue-Description:
//
// SimplexSolver throws UnboundedSolutionException when trying to solve minimization linear programming problem. The number of exception thrown depends on the number of variables.
//
//
// In order to see that behavior of SimplexSolver first try to run JUnit test setting a final variable ENTITIES\_COUNT = 2 and that will give almost good result and then set it to 15 and you'll get a massive of unbounded exceptions.
//
// First iteration is runned with predefined set of input data with which the Solver gives back an appropriate result.
//
//
// The problem itself is well tested by it's authors (mathematicians who I believe know what they developed) using Matlab 10 with no unbounded solutions on the same rules of creatnig random variables values.
//
//
// What is strange to me is the dependence of the number of UnboundedSolutionException exceptions on the number of variables in the problem.
//
//
// The problem is formulated as
//
// min(1\*t + 0\*L) (for every r-th subject)
//
// s.t.
//
// -q(r) + QL >= 0
//
// x(r)t - XL >= 0
//
// L >= 0
//
// where
//
// r = 1..R,
//
// L =
//
//
// {l(1), l(2), ..., l(R)}
// (vector of R rows and 1 column),
//
// Q - coefficients matrix MxR
//
// X - coefficients matrix NxR
//
//
//
//
//
@Test
public void testMath828Cycle() {
| 72 | 28 | 53 | src/test/java/org/apache/commons/math3/optimization/linear/SimplexSolverTest.java | src/test/java | ```markdown
## Issue-ID: Math-MATH-828
## Issue-Title:
Not expected UnboundedSolutionException
## Issue-Description:
SimplexSolver throws UnboundedSolutionException when trying to solve minimization linear programming problem. The number of exception thrown depends on the number of variables.
In order to see that behavior of SimplexSolver first try to run JUnit test setting a final variable ENTITIES\_COUNT = 2 and that will give almost good result and then set it to 15 and you'll get a massive of unbounded exceptions.
First iteration is runned with predefined set of input data with which the Solver gives back an appropriate result.
The problem itself is well tested by it's authors (mathematicians who I believe know what they developed) using Matlab 10 with no unbounded solutions on the same rules of creatnig random variables values.
What is strange to me is the dependence of the number of UnboundedSolutionException exceptions on the number of variables in the problem.
The problem is formulated as
min(1\*t + 0\*L) (for every r-th subject)
s.t.
-q(r) + QL >= 0
x(r)t - XL >= 0
L >= 0
where
r = 1..R,
L =
{l(1), l(2), ..., l(R)}
(vector of R rows and 1 column),
Q - coefficients matrix MxR
X - coefficients matrix NxR
```
You are a professional Java test case writer, please create a test case named `testMath828Cycle` for the issue `Math-MATH-828`, utilizing the provided issue report information and the following function signature.
```java
@Test
public void testMath828Cycle() {
```
| 53 | [
"org.apache.commons.math3.optimization.linear.SimplexSolver"
] | 843a5fe43db60c40b4957f79e3ca8cc4a70bbe09de493298cc8dc0e53652214a | @Test
public void testMath828Cycle() | // You are a professional Java test case writer, please create a test case named `testMath828Cycle` for the issue `Math-MATH-828`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Math-MATH-828
//
// ## Issue-Title:
// Not expected UnboundedSolutionException
//
// ## Issue-Description:
//
// SimplexSolver throws UnboundedSolutionException when trying to solve minimization linear programming problem. The number of exception thrown depends on the number of variables.
//
//
// In order to see that behavior of SimplexSolver first try to run JUnit test setting a final variable ENTITIES\_COUNT = 2 and that will give almost good result and then set it to 15 and you'll get a massive of unbounded exceptions.
//
// First iteration is runned with predefined set of input data with which the Solver gives back an appropriate result.
//
//
// The problem itself is well tested by it's authors (mathematicians who I believe know what they developed) using Matlab 10 with no unbounded solutions on the same rules of creatnig random variables values.
//
//
// What is strange to me is the dependence of the number of UnboundedSolutionException exceptions on the number of variables in the problem.
//
//
// The problem is formulated as
//
// min(1\*t + 0\*L) (for every r-th subject)
//
// s.t.
//
// -q(r) + QL >= 0
//
// x(r)t - XL >= 0
//
// L >= 0
//
// where
//
// r = 1..R,
//
// L =
//
//
// {l(1), l(2), ..., l(R)}
// (vector of R rows and 1 column),
//
// Q - coefficients matrix MxR
//
// X - coefficients matrix NxR
//
//
//
//
//
| Math | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.math3.optimization.linear;
import org.junit.Assert;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import org.apache.commons.math3.optimization.GoalType;
import org.apache.commons.math3.optimization.PointValuePair;
import org.apache.commons.math3.util.Precision;
import org.junit.Test;
public class SimplexSolverTest {
@Test
public void testMath828() {
LinearObjectiveFunction f = new LinearObjectiveFunction(
new double[] { 1.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0}, 0.0);
ArrayList <LinearConstraint>constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] {0.0, 39.0, 23.0, 96.0, 15.0, 48.0, 9.0, 21.0, 48.0, 36.0, 76.0, 19.0, 88.0, 17.0, 16.0, 36.0,}, Relationship.GEQ, 15.0));
constraints.add(new LinearConstraint(new double[] {0.0, 59.0, 93.0, 12.0, 29.0, 78.0, 73.0, 87.0, 32.0, 70.0, 68.0, 24.0, 11.0, 26.0, 65.0, 25.0,}, Relationship.GEQ, 29.0));
constraints.add(new LinearConstraint(new double[] {0.0, 74.0, 5.0, 82.0, 6.0, 97.0, 55.0, 44.0, 52.0, 54.0, 5.0, 93.0, 91.0, 8.0, 20.0, 97.0,}, Relationship.GEQ, 6.0));
constraints.add(new LinearConstraint(new double[] {8.0, -3.0, -28.0, -72.0, -8.0, -31.0, -31.0, -74.0, -47.0, -59.0, -24.0, -57.0, -56.0, -16.0, -92.0, -59.0,}, Relationship.GEQ, 0.0));
constraints.add(new LinearConstraint(new double[] {25.0, -7.0, -99.0, -78.0, -25.0, -14.0, -16.0, -89.0, -39.0, -56.0, -53.0, -9.0, -18.0, -26.0, -11.0, -61.0,}, Relationship.GEQ, 0.0));
constraints.add(new LinearConstraint(new double[] {33.0, -95.0, -15.0, -4.0, -33.0, -3.0, -20.0, -96.0, -27.0, -13.0, -80.0, -24.0, -3.0, -13.0, -57.0, -76.0,}, Relationship.GEQ, 0.0));
constraints.add(new LinearConstraint(new double[] {7.0, -95.0, -39.0, -93.0, -7.0, -94.0, -94.0, -62.0, -76.0, -26.0, -53.0, -57.0, -31.0, -76.0, -53.0, -52.0,}, Relationship.GEQ, 0.0));
double epsilon = 1e-6;
PointValuePair solution = new SimplexSolver().optimize(f, constraints, GoalType.MINIMIZE, true);
Assert.assertEquals(1.0d, solution.getValue(), epsilon);
Assert.assertTrue(validSolution(solution, constraints, epsilon));
}
@Test
public void testMath828Cycle() {
LinearObjectiveFunction f = new LinearObjectiveFunction(
new double[] { 1.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0}, 0.0);
ArrayList <LinearConstraint>constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] {0.0, 16.0, 14.0, 69.0, 1.0, 85.0, 52.0, 43.0, 64.0, 97.0, 14.0, 74.0, 89.0, 28.0, 94.0, 58.0, 13.0, 22.0, 21.0, 17.0, 30.0, 25.0, 1.0, 59.0, 91.0, 78.0, 12.0, 74.0, 56.0, 3.0, 88.0,}, Relationship.GEQ, 91.0));
constraints.add(new LinearConstraint(new double[] {0.0, 60.0, 40.0, 81.0, 71.0, 72.0, 46.0, 45.0, 38.0, 48.0, 40.0, 17.0, 33.0, 85.0, 64.0, 32.0, 84.0, 3.0, 54.0, 44.0, 71.0, 67.0, 90.0, 95.0, 54.0, 99.0, 99.0, 29.0, 52.0, 98.0, 9.0,}, Relationship.GEQ, 54.0));
constraints.add(new LinearConstraint(new double[] {0.0, 41.0, 12.0, 86.0, 90.0, 61.0, 31.0, 41.0, 23.0, 89.0, 17.0, 74.0, 44.0, 27.0, 16.0, 47.0, 80.0, 32.0, 11.0, 56.0, 68.0, 82.0, 11.0, 62.0, 62.0, 53.0, 39.0, 16.0, 48.0, 1.0, 63.0,}, Relationship.GEQ, 62.0));
constraints.add(new LinearConstraint(new double[] {83.0, -76.0, -94.0, -19.0, -15.0, -70.0, -72.0, -57.0, -63.0, -65.0, -22.0, -94.0, -22.0, -88.0, -86.0, -89.0, -72.0, -16.0, -80.0, -49.0, -70.0, -93.0, -95.0, -17.0, -83.0, -97.0, -31.0, -47.0, -31.0, -13.0, -23.0,}, Relationship.GEQ, 0.0));
constraints.add(new LinearConstraint(new double[] {41.0, -96.0, -41.0, -48.0, -70.0, -43.0, -43.0, -43.0, -97.0, -37.0, -85.0, -70.0, -45.0, -67.0, -87.0, -69.0, -94.0, -54.0, -54.0, -92.0, -79.0, -10.0, -35.0, -20.0, -41.0, -41.0, -65.0, -25.0, -12.0, -8.0, -46.0,}, Relationship.GEQ, 0.0));
constraints.add(new LinearConstraint(new double[] {27.0, -42.0, -65.0, -49.0, -53.0, -42.0, -17.0, -2.0, -61.0, -31.0, -76.0, -47.0, -8.0, -93.0, -86.0, -62.0, -65.0, -63.0, -22.0, -43.0, -27.0, -23.0, -32.0, -74.0, -27.0, -63.0, -47.0, -78.0, -29.0, -95.0, -73.0,}, Relationship.GEQ, 0.0));
constraints.add(new LinearConstraint(new double[] {15.0, -46.0, -41.0, -83.0, -98.0, -99.0, -21.0, -35.0, -7.0, -14.0, -80.0, -63.0, -18.0, -42.0, -5.0, -34.0, -56.0, -70.0, -16.0, -18.0, -74.0, -61.0, -47.0, -41.0, -15.0, -79.0, -18.0, -47.0, -88.0, -68.0, -55.0,}, Relationship.GEQ, 0.0));
double epsilon = 1e-6;
PointValuePair solution = new SimplexSolver().optimize(f, constraints, GoalType.MINIMIZE, true);
Assert.assertEquals(1.0d, solution.getValue(), epsilon);
Assert.assertTrue(validSolution(solution, constraints, epsilon));
}
@Test
public void testMath781() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 2, 6, 7 }, 0);
ArrayList<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 2, 1 }, Relationship.LEQ, 2));
constraints.add(new LinearConstraint(new double[] { -1, 1, 1 }, Relationship.LEQ, -1));
constraints.add(new LinearConstraint(new double[] { 2, -3, 1 }, Relationship.LEQ, -1));
double epsilon = 1e-6;
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, false);
Assert.assertTrue(Precision.compareTo(solution.getPoint()[0], 0.0d, epsilon) > 0);
Assert.assertTrue(Precision.compareTo(solution.getPoint()[1], 0.0d, epsilon) > 0);
Assert.assertTrue(Precision.compareTo(solution.getPoint()[2], 0.0d, epsilon) < 0);
Assert.assertEquals(2.0d, solution.getValue(), epsilon);
}
@Test
public void testMath713NegativeVariable() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] {1.0, 1.0}, 0.0d);
ArrayList<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] {1, 0}, Relationship.EQ, 1));
double epsilon = 1e-6;
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MINIMIZE, true);
Assert.assertTrue(Precision.compareTo(solution.getPoint()[0], 0.0d, epsilon) >= 0);
Assert.assertTrue(Precision.compareTo(solution.getPoint()[1], 0.0d, epsilon) >= 0);
}
@Test
public void testMath434NegativeVariable() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] {0.0, 0.0, 1.0}, 0.0d);
ArrayList<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] {1, 1, 0}, Relationship.EQ, 5));
constraints.add(new LinearConstraint(new double[] {0, 0, 1}, Relationship.GEQ, -10));
double epsilon = 1e-6;
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MINIMIZE, false);
Assert.assertEquals(5.0, solution.getPoint()[0] + solution.getPoint()[1], epsilon);
Assert.assertEquals(-10.0, solution.getPoint()[2], epsilon);
Assert.assertEquals(-10.0, solution.getValue(), epsilon);
}
@Test(expected = NoFeasibleSolutionException.class)
public void testMath434UnfeasibleSolution() {
double epsilon = 1e-6;
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] {1.0, 0.0}, 0.0);
ArrayList<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] {epsilon/2, 0.5}, Relationship.EQ, 0));
constraints.add(new LinearConstraint(new double[] {1e-3, 0.1}, Relationship.EQ, 10));
SimplexSolver solver = new SimplexSolver();
// allowing only non-negative values, no feasible solution shall be found
solver.optimize(f, constraints, GoalType.MINIMIZE, true);
}
@Test
public void testMath434PivotRowSelection() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] {1.0}, 0.0);
double epsilon = 1e-6;
ArrayList<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] {200}, Relationship.GEQ, 1));
constraints.add(new LinearConstraint(new double[] {100}, Relationship.GEQ, 0.499900001));
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MINIMIZE, false);
Assert.assertTrue(Precision.compareTo(solution.getPoint()[0] * 200.d, 1.d, epsilon) >= 0);
Assert.assertEquals(0.0050, solution.getValue(), epsilon);
}
@Test
public void testMath434PivotRowSelection2() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] {0.0d, 1.0d, 1.0d, 0.0d, 0.0d, 0.0d, 0.0d}, 0.0d);
ArrayList<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] {1.0d, -0.1d, 0.0d, 0.0d, 0.0d, 0.0d, 0.0d}, Relationship.EQ, -0.1d));
constraints.add(new LinearConstraint(new double[] {1.0d, 0.0d, 0.0d, 0.0d, 0.0d, 0.0d, 0.0d}, Relationship.GEQ, -1e-18d));
constraints.add(new LinearConstraint(new double[] {0.0d, 1.0d, 0.0d, 0.0d, 0.0d, 0.0d, 0.0d}, Relationship.GEQ, 0.0d));
constraints.add(new LinearConstraint(new double[] {0.0d, 0.0d, 0.0d, 1.0d, 0.0d, -0.0128588d, 1e-5d}, Relationship.EQ, 0.0d));
constraints.add(new LinearConstraint(new double[] {0.0d, 0.0d, 0.0d, 0.0d, 1.0d, 1e-5d, -0.0128586d}, Relationship.EQ, 1e-10d));
constraints.add(new LinearConstraint(new double[] {0.0d, 0.0d, 1.0d, -1.0d, 0.0d, 0.0d, 0.0d}, Relationship.GEQ, 0.0d));
constraints.add(new LinearConstraint(new double[] {0.0d, 0.0d, 1.0d, 1.0d, 0.0d, 0.0d, 0.0d}, Relationship.GEQ, 0.0d));
constraints.add(new LinearConstraint(new double[] {0.0d, 0.0d, 1.0d, 0.0d, -1.0d, 0.0d, 0.0d}, Relationship.GEQ, 0.0d));
constraints.add(new LinearConstraint(new double[] {0.0d, 0.0d, 1.0d, 0.0d, 1.0d, 0.0d, 0.0d}, Relationship.GEQ, 0.0d));
double epsilon = 1e-7;
SimplexSolver simplex = new SimplexSolver();
PointValuePair solution = simplex.optimize(f, constraints, GoalType.MINIMIZE, false);
Assert.assertTrue(Precision.compareTo(solution.getPoint()[0], -1e-18d, epsilon) >= 0);
Assert.assertEquals(1.0d, solution.getPoint()[1], epsilon);
Assert.assertEquals(0.0d, solution.getPoint()[2], epsilon);
Assert.assertEquals(1.0d, solution.getValue(), epsilon);
}
@Test
public void testMath272() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 2, 2, 1 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 1, 0 }, Relationship.GEQ, 1));
constraints.add(new LinearConstraint(new double[] { 1, 0, 1 }, Relationship.GEQ, 1));
constraints.add(new LinearConstraint(new double[] { 0, 1, 0 }, Relationship.GEQ, 1));
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MINIMIZE, true);
Assert.assertEquals(0.0, solution.getPoint()[0], .0000001);
Assert.assertEquals(1.0, solution.getPoint()[1], .0000001);
Assert.assertEquals(1.0, solution.getPoint()[2], .0000001);
Assert.assertEquals(3.0, solution.getValue(), .0000001);
}
@Test
public void testMath286() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 0.8, 0.2, 0.7, 0.3, 0.6, 0.4 }, 0 );
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 0, 1, 0, 1, 0 }, Relationship.EQ, 23.0));
constraints.add(new LinearConstraint(new double[] { 0, 1, 0, 1, 0, 1 }, Relationship.EQ, 23.0));
constraints.add(new LinearConstraint(new double[] { 1, 0, 0, 0, 0, 0 }, Relationship.GEQ, 10.0));
constraints.add(new LinearConstraint(new double[] { 0, 0, 1, 0, 0, 0 }, Relationship.GEQ, 8.0));
constraints.add(new LinearConstraint(new double[] { 0, 0, 0, 0, 1, 0 }, Relationship.GEQ, 5.0));
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, true);
Assert.assertEquals(25.8, solution.getValue(), .0000001);
Assert.assertEquals(23.0, solution.getPoint()[0] + solution.getPoint()[2] + solution.getPoint()[4], 0.0000001);
Assert.assertEquals(23.0, solution.getPoint()[1] + solution.getPoint()[3] + solution.getPoint()[5], 0.0000001);
Assert.assertTrue(solution.getPoint()[0] >= 10.0 - 0.0000001);
Assert.assertTrue(solution.getPoint()[2] >= 8.0 - 0.0000001);
Assert.assertTrue(solution.getPoint()[4] >= 5.0 - 0.0000001);
}
@Test
public void testDegeneracy() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 0.8, 0.7 }, 0 );
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 1 }, Relationship.LEQ, 18.0));
constraints.add(new LinearConstraint(new double[] { 1, 0 }, Relationship.GEQ, 10.0));
constraints.add(new LinearConstraint(new double[] { 0, 1 }, Relationship.GEQ, 8.0));
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, true);
Assert.assertEquals(13.6, solution.getValue(), .0000001);
}
@Test
public void testMath288() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 7, 3, 0, 0 }, 0 );
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 3, 0, -5, 0 }, Relationship.LEQ, 0.0));
constraints.add(new LinearConstraint(new double[] { 2, 0, 0, -5 }, Relationship.LEQ, 0.0));
constraints.add(new LinearConstraint(new double[] { 0, 3, 0, -5 }, Relationship.LEQ, 0.0));
constraints.add(new LinearConstraint(new double[] { 1, 0, 0, 0 }, Relationship.LEQ, 1.0));
constraints.add(new LinearConstraint(new double[] { 0, 1, 0, 0 }, Relationship.LEQ, 1.0));
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, true);
Assert.assertEquals(10.0, solution.getValue(), .0000001);
}
@Test
public void testMath290GEQ() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 1, 5 }, 0 );
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 2, 0 }, Relationship.GEQ, -1.0));
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MINIMIZE, true);
Assert.assertEquals(0, solution.getValue(), .0000001);
Assert.assertEquals(0, solution.getPoint()[0], .0000001);
Assert.assertEquals(0, solution.getPoint()[1], .0000001);
}
@Test(expected=NoFeasibleSolutionException.class)
public void testMath290LEQ() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 1, 5 }, 0 );
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 2, 0 }, Relationship.LEQ, -1.0));
SimplexSolver solver = new SimplexSolver();
solver.optimize(f, constraints, GoalType.MINIMIZE, true);
}
@Test
public void testMath293() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 0.8, 0.2, 0.7, 0.3, 0.4, 0.6}, 0 );
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 0, 1, 0, 1, 0 }, Relationship.EQ, 30.0));
constraints.add(new LinearConstraint(new double[] { 0, 1, 0, 1, 0, 1 }, Relationship.EQ, 30.0));
constraints.add(new LinearConstraint(new double[] { 0.8, 0.2, 0.0, 0.0, 0.0, 0.0 }, Relationship.GEQ, 10.0));
constraints.add(new LinearConstraint(new double[] { 0.0, 0.0, 0.7, 0.3, 0.0, 0.0 }, Relationship.GEQ, 10.0));
constraints.add(new LinearConstraint(new double[] { 0.0, 0.0, 0.0, 0.0, 0.4, 0.6 }, Relationship.GEQ, 10.0));
SimplexSolver solver = new SimplexSolver();
PointValuePair solution1 = solver.optimize(f, constraints, GoalType.MAXIMIZE, true);
Assert.assertEquals(15.7143, solution1.getPoint()[0], .0001);
Assert.assertEquals(0.0, solution1.getPoint()[1], .0001);
Assert.assertEquals(14.2857, solution1.getPoint()[2], .0001);
Assert.assertEquals(0.0, solution1.getPoint()[3], .0001);
Assert.assertEquals(0.0, solution1.getPoint()[4], .0001);
Assert.assertEquals(30.0, solution1.getPoint()[5], .0001);
Assert.assertEquals(40.57143, solution1.getValue(), .0001);
double valA = 0.8 * solution1.getPoint()[0] + 0.2 * solution1.getPoint()[1];
double valB = 0.7 * solution1.getPoint()[2] + 0.3 * solution1.getPoint()[3];
double valC = 0.4 * solution1.getPoint()[4] + 0.6 * solution1.getPoint()[5];
f = new LinearObjectiveFunction(new double[] { 0.8, 0.2, 0.7, 0.3, 0.4, 0.6}, 0 );
constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 0, 1, 0, 1, 0 }, Relationship.EQ, 30.0));
constraints.add(new LinearConstraint(new double[] { 0, 1, 0, 1, 0, 1 }, Relationship.EQ, 30.0));
constraints.add(new LinearConstraint(new double[] { 0.8, 0.2, 0.0, 0.0, 0.0, 0.0 }, Relationship.GEQ, valA));
constraints.add(new LinearConstraint(new double[] { 0.0, 0.0, 0.7, 0.3, 0.0, 0.0 }, Relationship.GEQ, valB));
constraints.add(new LinearConstraint(new double[] { 0.0, 0.0, 0.0, 0.0, 0.4, 0.6 }, Relationship.GEQ, valC));
PointValuePair solution2 = solver.optimize(f, constraints, GoalType.MAXIMIZE, true);
Assert.assertEquals(40.57143, solution2.getValue(), .0001);
}
@Test
public void testSimplexSolver() {
LinearObjectiveFunction f =
new LinearObjectiveFunction(new double[] { 15, 10 }, 7);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 0 }, Relationship.LEQ, 2));
constraints.add(new LinearConstraint(new double[] { 0, 1 }, Relationship.LEQ, 3));
constraints.add(new LinearConstraint(new double[] { 1, 1 }, Relationship.EQ, 4));
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, false);
Assert.assertEquals(2.0, solution.getPoint()[0], 0.0);
Assert.assertEquals(2.0, solution.getPoint()[1], 0.0);
Assert.assertEquals(57.0, solution.getValue(), 0.0);
}
@Test
public void testSingleVariableAndConstraint() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 3 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1 }, Relationship.LEQ, 10));
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, false);
Assert.assertEquals(10.0, solution.getPoint()[0], 0.0);
Assert.assertEquals(30.0, solution.getValue(), 0.0);
}
/**
* With no artificial variables needed (no equals and no greater than
* constraints) we can go straight to Phase 2.
*/
@Test
public void testModelWithNoArtificialVars() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 15, 10 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 0 }, Relationship.LEQ, 2));
constraints.add(new LinearConstraint(new double[] { 0, 1 }, Relationship.LEQ, 3));
constraints.add(new LinearConstraint(new double[] { 1, 1 }, Relationship.LEQ, 4));
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, false);
Assert.assertEquals(2.0, solution.getPoint()[0], 0.0);
Assert.assertEquals(2.0, solution.getPoint()[1], 0.0);
Assert.assertEquals(50.0, solution.getValue(), 0.0);
}
@Test
public void testMinimization() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { -2, 1 }, -5);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 2 }, Relationship.LEQ, 6));
constraints.add(new LinearConstraint(new double[] { 3, 2 }, Relationship.LEQ, 12));
constraints.add(new LinearConstraint(new double[] { 0, 1 }, Relationship.GEQ, 0));
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MINIMIZE, false);
Assert.assertEquals(4.0, solution.getPoint()[0], 0.0);
Assert.assertEquals(0.0, solution.getPoint()[1], 0.0);
Assert.assertEquals(-13.0, solution.getValue(), 0.0);
}
@Test
public void testSolutionWithNegativeDecisionVariable() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { -2, 1 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 1 }, Relationship.GEQ, 6));
constraints.add(new LinearConstraint(new double[] { 1, 2 }, Relationship.LEQ, 14));
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, false);
Assert.assertEquals(-2.0, solution.getPoint()[0], 0.0);
Assert.assertEquals(8.0, solution.getPoint()[1], 0.0);
Assert.assertEquals(12.0, solution.getValue(), 0.0);
}
@Test(expected = NoFeasibleSolutionException.class)
public void testInfeasibleSolution() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 15 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1 }, Relationship.LEQ, 1));
constraints.add(new LinearConstraint(new double[] { 1 }, Relationship.GEQ, 3));
SimplexSolver solver = new SimplexSolver();
solver.optimize(f, constraints, GoalType.MAXIMIZE, false);
}
@Test(expected = UnboundedSolutionException.class)
public void testUnboundedSolution() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 15, 10 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 0 }, Relationship.EQ, 2));
SimplexSolver solver = new SimplexSolver();
solver.optimize(f, constraints, GoalType.MAXIMIZE, false);
}
@Test
public void testRestrictVariablesToNonNegative() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 409, 523, 70, 204, 339 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 43, 56, 345, 56, 5 }, Relationship.LEQ, 4567456));
constraints.add(new LinearConstraint(new double[] { 12, 45, 7, 56, 23 }, Relationship.LEQ, 56454));
constraints.add(new LinearConstraint(new double[] { 8, 768, 0, 34, 7456 }, Relationship.LEQ, 1923421));
constraints.add(new LinearConstraint(new double[] { 12342, 2342, 34, 678, 2342 }, Relationship.GEQ, 4356));
constraints.add(new LinearConstraint(new double[] { 45, 678, 76, 52, 23 }, Relationship.EQ, 456356));
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, true);
Assert.assertEquals(2902.92783505155, solution.getPoint()[0], .0000001);
Assert.assertEquals(480.419243986254, solution.getPoint()[1], .0000001);
Assert.assertEquals(0.0, solution.getPoint()[2], .0000001);
Assert.assertEquals(0.0, solution.getPoint()[3], .0000001);
Assert.assertEquals(0.0, solution.getPoint()[4], .0000001);
Assert.assertEquals(1438556.7491409, solution.getValue(), .0000001);
}
@Test
public void testEpsilon() {
LinearObjectiveFunction f =
new LinearObjectiveFunction(new double[] { 10, 5, 1 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 9, 8, 0 }, Relationship.EQ, 17));
constraints.add(new LinearConstraint(new double[] { 0, 7, 8 }, Relationship.LEQ, 7));
constraints.add(new LinearConstraint(new double[] { 10, 0, 2 }, Relationship.LEQ, 10));
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, false);
Assert.assertEquals(1.0, solution.getPoint()[0], 0.0);
Assert.assertEquals(1.0, solution.getPoint()[1], 0.0);
Assert.assertEquals(0.0, solution.getPoint()[2], 0.0);
Assert.assertEquals(15.0, solution.getValue(), 0.0);
}
@Test
public void testTrivialModel() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 1, 1 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 1 }, Relationship.EQ, 0));
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, true);
Assert.assertEquals(0, solution.getValue(), .0000001);
}
@Test
public void testLargeModel() {
double[] objective = new double[] {
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 12, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
12, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 12, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 12, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 12, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 12, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1};
LinearObjectiveFunction f = new LinearObjectiveFunction(objective, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(equationFromString(objective.length, "x0 + x1 + x2 + x3 - x12 = 0"));
constraints.add(equationFromString(objective.length, "x4 + x5 + x6 + x7 + x8 + x9 + x10 + x11 - x13 = 0"));
constraints.add(equationFromString(objective.length, "x4 + x5 + x6 + x7 + x8 + x9 + x10 + x11 >= 49"));
constraints.add(equationFromString(objective.length, "x0 + x1 + x2 + x3 >= 42"));
constraints.add(equationFromString(objective.length, "x14 + x15 + x16 + x17 - x26 = 0"));
constraints.add(equationFromString(objective.length, "x18 + x19 + x20 + x21 + x22 + x23 + x24 + x25 - x27 = 0"));
constraints.add(equationFromString(objective.length, "x14 + x15 + x16 + x17 - x12 = 0"));
constraints.add(equationFromString(objective.length, "x18 + x19 + x20 + x21 + x22 + x23 + x24 + x25 - x13 = 0"));
constraints.add(equationFromString(objective.length, "x28 + x29 + x30 + x31 - x40 = 0"));
constraints.add(equationFromString(objective.length, "x32 + x33 + x34 + x35 + x36 + x37 + x38 + x39 - x41 = 0"));
constraints.add(equationFromString(objective.length, "x32 + x33 + x34 + x35 + x36 + x37 + x38 + x39 >= 49"));
constraints.add(equationFromString(objective.length, "x28 + x29 + x30 + x31 >= 42"));
constraints.add(equationFromString(objective.length, "x42 + x43 + x44 + x45 - x54 = 0"));
constraints.add(equationFromString(objective.length, "x46 + x47 + x48 + x49 + x50 + x51 + x52 + x53 - x55 = 0"));
constraints.add(equationFromString(objective.length, "x42 + x43 + x44 + x45 - x40 = 0"));
constraints.add(equationFromString(objective.length, "x46 + x47 + x48 + x49 + x50 + x51 + x52 + x53 - x41 = 0"));
constraints.add(equationFromString(objective.length, "x56 + x57 + x58 + x59 - x68 = 0"));
constraints.add(equationFromString(objective.length, "x60 + x61 + x62 + x63 + x64 + x65 + x66 + x67 - x69 = 0"));
constraints.add(equationFromString(objective.length, "x60 + x61 + x62 + x63 + x64 + x65 + x66 + x67 >= 51"));
constraints.add(equationFromString(objective.length, "x56 + x57 + x58 + x59 >= 44"));
constraints.add(equationFromString(objective.length, "x70 + x71 + x72 + x73 - x82 = 0"));
constraints.add(equationFromString(objective.length, "x74 + x75 + x76 + x77 + x78 + x79 + x80 + x81 - x83 = 0"));
constraints.add(equationFromString(objective.length, "x70 + x71 + x72 + x73 - x68 = 0"));
constraints.add(equationFromString(objective.length, "x74 + x75 + x76 + x77 + x78 + x79 + x80 + x81 - x69 = 0"));
constraints.add(equationFromString(objective.length, "x84 + x85 + x86 + x87 - x96 = 0"));
constraints.add(equationFromString(objective.length, "x88 + x89 + x90 + x91 + x92 + x93 + x94 + x95 - x97 = 0"));
constraints.add(equationFromString(objective.length, "x88 + x89 + x90 + x91 + x92 + x93 + x94 + x95 >= 51"));
constraints.add(equationFromString(objective.length, "x84 + x85 + x86 + x87 >= 44"));
constraints.add(equationFromString(objective.length, "x98 + x99 + x100 + x101 - x110 = 0"));
constraints.add(equationFromString(objective.length, "x102 + x103 + x104 + x105 + x106 + x107 + x108 + x109 - x111 = 0"));
constraints.add(equationFromString(objective.length, "x98 + x99 + x100 + x101 - x96 = 0"));
constraints.add(equationFromString(objective.length, "x102 + x103 + x104 + x105 + x106 + x107 + x108 + x109 - x97 = 0"));
constraints.add(equationFromString(objective.length, "x112 + x113 + x114 + x115 - x124 = 0"));
constraints.add(equationFromString(objective.length, "x116 + x117 + x118 + x119 + x120 + x121 + x122 + x123 - x125 = 0"));
constraints.add(equationFromString(objective.length, "x116 + x117 + x118 + x119 + x120 + x121 + x122 + x123 >= 49"));
constraints.add(equationFromString(objective.length, "x112 + x113 + x114 + x115 >= 42"));
constraints.add(equationFromString(objective.length, "x126 + x127 + x128 + x129 - x138 = 0"));
constraints.add(equationFromString(objective.length, "x130 + x131 + x132 + x133 + x134 + x135 + x136 + x137 - x139 = 0"));
constraints.add(equationFromString(objective.length, "x126 + x127 + x128 + x129 - x124 = 0"));
constraints.add(equationFromString(objective.length, "x130 + x131 + x132 + x133 + x134 + x135 + x136 + x137 - x125 = 0"));
constraints.add(equationFromString(objective.length, "x140 + x141 + x142 + x143 - x152 = 0"));
constraints.add(equationFromString(objective.length, "x144 + x145 + x146 + x147 + x148 + x149 + x150 + x151 - x153 = 0"));
constraints.add(equationFromString(objective.length, "x144 + x145 + x146 + x147 + x148 + x149 + x150 + x151 >= 59"));
constraints.add(equationFromString(objective.length, "x140 + x141 + x142 + x143 >= 42"));
constraints.add(equationFromString(objective.length, "x154 + x155 + x156 + x157 - x166 = 0"));
constraints.add(equationFromString(objective.length, "x158 + x159 + x160 + x161 + x162 + x163 + x164 + x165 - x167 = 0"));
constraints.add(equationFromString(objective.length, "x154 + x155 + x156 + x157 - x152 = 0"));
constraints.add(equationFromString(objective.length, "x158 + x159 + x160 + x161 + x162 + x163 + x164 + x165 - x153 = 0"));
constraints.add(equationFromString(objective.length, "x83 + x82 - x168 = 0"));
constraints.add(equationFromString(objective.length, "x111 + x110 - x169 = 0"));
constraints.add(equationFromString(objective.length, "x170 - x182 = 0"));
constraints.add(equationFromString(objective.length, "x171 - x183 = 0"));
constraints.add(equationFromString(objective.length, "x172 - x184 = 0"));
constraints.add(equationFromString(objective.length, "x173 - x185 = 0"));
constraints.add(equationFromString(objective.length, "x174 - x186 = 0"));
constraints.add(equationFromString(objective.length, "x175 + x176 - x187 = 0"));
constraints.add(equationFromString(objective.length, "x177 - x188 = 0"));
constraints.add(equationFromString(objective.length, "x178 - x189 = 0"));
constraints.add(equationFromString(objective.length, "x179 - x190 = 0"));
constraints.add(equationFromString(objective.length, "x180 - x191 = 0"));
constraints.add(equationFromString(objective.length, "x181 - x192 = 0"));
constraints.add(equationFromString(objective.length, "x170 - x26 = 0"));
constraints.add(equationFromString(objective.length, "x171 - x27 = 0"));
constraints.add(equationFromString(objective.length, "x172 - x54 = 0"));
constraints.add(equationFromString(objective.length, "x173 - x55 = 0"));
constraints.add(equationFromString(objective.length, "x174 - x168 = 0"));
constraints.add(equationFromString(objective.length, "x177 - x169 = 0"));
constraints.add(equationFromString(objective.length, "x178 - x138 = 0"));
constraints.add(equationFromString(objective.length, "x179 - x139 = 0"));
constraints.add(equationFromString(objective.length, "x180 - x166 = 0"));
constraints.add(equationFromString(objective.length, "x181 - x167 = 0"));
constraints.add(equationFromString(objective.length, "x193 - x205 = 0"));
constraints.add(equationFromString(objective.length, "x194 - x206 = 0"));
constraints.add(equationFromString(objective.length, "x195 - x207 = 0"));
constraints.add(equationFromString(objective.length, "x196 - x208 = 0"));
constraints.add(equationFromString(objective.length, "x197 - x209 = 0"));
constraints.add(equationFromString(objective.length, "x198 + x199 - x210 = 0"));
constraints.add(equationFromString(objective.length, "x200 - x211 = 0"));
constraints.add(equationFromString(objective.length, "x201 - x212 = 0"));
constraints.add(equationFromString(objective.length, "x202 - x213 = 0"));
constraints.add(equationFromString(objective.length, "x203 - x214 = 0"));
constraints.add(equationFromString(objective.length, "x204 - x215 = 0"));
constraints.add(equationFromString(objective.length, "x193 - x182 = 0"));
constraints.add(equationFromString(objective.length, "x194 - x183 = 0"));
constraints.add(equationFromString(objective.length, "x195 - x184 = 0"));
constraints.add(equationFromString(objective.length, "x196 - x185 = 0"));
constraints.add(equationFromString(objective.length, "x197 - x186 = 0"));
constraints.add(equationFromString(objective.length, "x198 + x199 - x187 = 0"));
constraints.add(equationFromString(objective.length, "x200 - x188 = 0"));
constraints.add(equationFromString(objective.length, "x201 - x189 = 0"));
constraints.add(equationFromString(objective.length, "x202 - x190 = 0"));
constraints.add(equationFromString(objective.length, "x203 - x191 = 0"));
constraints.add(equationFromString(objective.length, "x204 - x192 = 0"));
SimplexSolver solver = new SimplexSolver();
PointValuePair solution = solver.optimize(f, constraints, GoalType.MINIMIZE, true);
Assert.assertEquals(7518.0, solution.getValue(), .0000001);
}
/**
* Converts a test string to a {@link LinearConstraint}.
* Ex: x0 + x1 + x2 + x3 - x12 = 0
*/
private LinearConstraint equationFromString(int numCoefficients, String s) {
Relationship relationship;
if (s.contains(">=")) {
relationship = Relationship.GEQ;
} else if (s.contains("<=")) {
relationship = Relationship.LEQ;
} else if (s.contains("=")) {
relationship = Relationship.EQ;
} else {
throw new IllegalArgumentException();
}
String[] equationParts = s.split("[>|<]?=");
double rhs = Double.parseDouble(equationParts[1].trim());
double[] lhs = new double[numCoefficients];
String left = equationParts[0].replaceAll(" ?x", "");
String[] coefficients = left.split(" ");
for (String coefficient : coefficients) {
double value = coefficient.charAt(0) == '-' ? -1 : 1;
int index = Integer.parseInt(coefficient.replaceFirst("[+|-]", "").trim());
lhs[index] = value;
}
return new LinearConstraint(lhs, relationship, rhs);
}
private static boolean validSolution(PointValuePair solution, List<LinearConstraint> constraints, double epsilon) {
double[] vals = solution.getPoint();
for (LinearConstraint c : constraints) {
double[] coeffs = c.getCoefficients().toArray();
double result = 0.0d;
for (int i = 0; i < vals.length; i++) {
result += vals[i] * coeffs[i];
}
switch (c.getRelationship()) {
case EQ:
if (!Precision.equals(result, c.getValue(), epsilon)) {
return false;
}
break;
case GEQ:
if (Precision.compareTo(result, c.getValue(), epsilon) < 0) {
return false;
}
break;
case LEQ:
if (Precision.compareTo(result, c.getValue(), epsilon) > 0) {
return false;
}
break;
}
}
return true;
}
} |
||
@Test
public void workaroundForBrokenTimeHeader() throws Exception {
URL tar = getClass().getResource("/simple-aix-native-tar.tar");
TarArchiveInputStream in = null;
try {
in = new TarArchiveInputStream(new FileInputStream(new File(new URI(tar.toString()))));
TarArchiveEntry tae = in.getNextTarEntry();
tae = in.getNextTarEntry();
assertEquals("sample/link-to-txt-file.lnk", tae.getName());
assertEquals(new Date(0), tae.getLastModifiedDate());
assertTrue(tae.isSymbolicLink());
} finally {
if (in != null) {
in.close();
}
}
} | org.apache.commons.compress.archivers.tar.TarArchiveInputStreamTest::workaroundForBrokenTimeHeader | src/test/java/org/apache/commons/compress/archivers/tar/TarArchiveInputStreamTest.java | 65 | src/test/java/org/apache/commons/compress/archivers/tar/TarArchiveInputStreamTest.java | workaroundForBrokenTimeHeader | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package org.apache.commons.compress.archivers.tar;
import java.io.File;
import java.io.FileInputStream;
import java.io.StringReader;
import java.net.URI;
import java.net.URL;
import java.util.Date;
import java.util.Map;
import org.junit.Test;
import static org.junit.Assert.assertEquals;
import static org.junit.Assert.assertTrue;
public class TarArchiveInputStreamTest {
@Test
public void readSimplePaxHeader() throws Exception {
Map<String, String> headers = new TarArchiveInputStream(null)
.parsePaxHeaders(new StringReader("30 atime=1321711775.972059463\n"));
assertEquals(1, headers.size());
assertEquals("1321711775.972059463", headers.get("atime"));
}
@Test
public void readPaxHeaderWithEmbeddedNewline() throws Exception {
Map<String, String> headers = new TarArchiveInputStream(null)
.parsePaxHeaders(new StringReader("28 comment=line1\nline2\nand3\n"));
assertEquals(1, headers.size());
assertEquals("line1\nline2\nand3", headers.get("comment"));
}
@Test
public void workaroundForBrokenTimeHeader() throws Exception {
URL tar = getClass().getResource("/simple-aix-native-tar.tar");
TarArchiveInputStream in = null;
try {
in = new TarArchiveInputStream(new FileInputStream(new File(new URI(tar.toString()))));
TarArchiveEntry tae = in.getNextTarEntry();
tae = in.getNextTarEntry();
assertEquals("sample/link-to-txt-file.lnk", tae.getName());
assertEquals(new Date(0), tae.getLastModifiedDate());
assertTrue(tae.isSymbolicLink());
} finally {
if (in != null) {
in.close();
}
}
}
} | // You are a professional Java test case writer, please create a test case named `workaroundForBrokenTimeHeader` for the issue `Compress-COMPRESS-181`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Compress-COMPRESS-181
//
// ## Issue-Title:
// Tar files created by AIX native tar, and which contain symlinks, cannot be read by TarArchiveInputStream
//
// ## Issue-Description:
//
// A simple tar file created on AIX using the native (/usr/bin/tar tar utility) **and** which contains a symbolic link, cannot be loaded by TarArchiveInputStream:
//
//
//
//
// ```
// java.io.IOException: Error detected parsing the header
// at org.apache.commons.compress.archivers.tar.TarArchiveInputStream.getNextTarEntry(TarArchiveInputStream.java:201)
// at Extractor.extract(Extractor.java:13)
// at Extractor.main(Extractor.java:28)
// at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
// at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:39)
// at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:25)
// at java.lang.reflect.Method.invoke(Method.java:597)
// at org.apache.tools.ant.taskdefs.ExecuteJava.run(ExecuteJava.java:217)
// at org.apache.tools.ant.taskdefs.ExecuteJava.execute(ExecuteJava.java:152)
// at org.apache.tools.ant.taskdefs.Java.run(Java.java:771)
// at org.apache.tools.ant.taskdefs.Java.executeJava(Java.java:221)
// at org.apache.tools.ant.taskdefs.Java.executeJava(Java.java:135)
// at org.apache.tools.ant.taskdefs.Java.execute(Java.java:108)
// at org.apache.tools.ant.UnknownElement.execute(UnknownElement.java:291)
// at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
// at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:39)
// at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:25)
// at java.lang.reflect.Method.invoke(Method.java:597)
// at org.apache.tools.ant.dispatch.DispatchUtils.execute(DispatchUtils.java:106)
// at org.apache.tools.ant.Task.perform(Task.java:348)
// at org.apache.tools.ant.Target.execute(Target.java:390)
// at org.apache.tools.ant.Target.performTasks(Target.java:411)
// at org.apache.tools.ant.Project.executeSortedTargets(Project.java:1399)
// at org.apache.tools.ant.Project.executeTarget(Project.java:1368)
// at org.apache.tools.ant.helper.DefaultExecutor.executeTargets(DefaultExecutor.java:41)
// at org.apache.tools.ant.Project.executeTargets(Project.java:1251)
// at org.apache.tools.ant.Main.runBuild(Main.java:809)
// at org.apache.tools.ant.Main.startAnt(Main.java:217)
// at org.apache.tools.ant.launch.Launcher.run(Launcher.java:280)
// at org.apache.tools.ant.launch.Launcher.main(Launcher.java:109)
// Caused by: java.lang.IllegalArgumentException: Invalid byte 0 at offset 0 in '{NUL}1722000726 ' len=12
// at org.apache.commons.compress.archivers.tar.TarUtils.parseOctal(TarUtils.java:99)
// at org.apache.commons.compress.archivers.tar.TarArchiveEntry.parseTarHeader(TarArchiveEntry.java:819)
// at org.apache.commons.compress.archivers.tar.TarArchiveEntry.<init>(TarArchiveEntry.java:314)
// at org.apache.commons.compress.archivers.tar.TarArchiveInputStream.getNextTarEntry(TarArchiveInputStream.java:199)
@Test
public void workaroundForBrokenTimeHeader() throws Exception {
| 65 | 14 | 49 | src/test/java/org/apache/commons/compress/archivers/tar/TarArchiveInputStreamTest.java | src/test/java | ```markdown
## Issue-ID: Compress-COMPRESS-181
## Issue-Title:
Tar files created by AIX native tar, and which contain symlinks, cannot be read by TarArchiveInputStream
## Issue-Description:
A simple tar file created on AIX using the native (/usr/bin/tar tar utility) **and** which contains a symbolic link, cannot be loaded by TarArchiveInputStream:
```
java.io.IOException: Error detected parsing the header
at org.apache.commons.compress.archivers.tar.TarArchiveInputStream.getNextTarEntry(TarArchiveInputStream.java:201)
at Extractor.extract(Extractor.java:13)
at Extractor.main(Extractor.java:28)
at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:39)
at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:25)
at java.lang.reflect.Method.invoke(Method.java:597)
at org.apache.tools.ant.taskdefs.ExecuteJava.run(ExecuteJava.java:217)
at org.apache.tools.ant.taskdefs.ExecuteJava.execute(ExecuteJava.java:152)
at org.apache.tools.ant.taskdefs.Java.run(Java.java:771)
at org.apache.tools.ant.taskdefs.Java.executeJava(Java.java:221)
at org.apache.tools.ant.taskdefs.Java.executeJava(Java.java:135)
at org.apache.tools.ant.taskdefs.Java.execute(Java.java:108)
at org.apache.tools.ant.UnknownElement.execute(UnknownElement.java:291)
at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:39)
at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:25)
at java.lang.reflect.Method.invoke(Method.java:597)
at org.apache.tools.ant.dispatch.DispatchUtils.execute(DispatchUtils.java:106)
at org.apache.tools.ant.Task.perform(Task.java:348)
at org.apache.tools.ant.Target.execute(Target.java:390)
at org.apache.tools.ant.Target.performTasks(Target.java:411)
at org.apache.tools.ant.Project.executeSortedTargets(Project.java:1399)
at org.apache.tools.ant.Project.executeTarget(Project.java:1368)
at org.apache.tools.ant.helper.DefaultExecutor.executeTargets(DefaultExecutor.java:41)
at org.apache.tools.ant.Project.executeTargets(Project.java:1251)
at org.apache.tools.ant.Main.runBuild(Main.java:809)
at org.apache.tools.ant.Main.startAnt(Main.java:217)
at org.apache.tools.ant.launch.Launcher.run(Launcher.java:280)
at org.apache.tools.ant.launch.Launcher.main(Launcher.java:109)
Caused by: java.lang.IllegalArgumentException: Invalid byte 0 at offset 0 in '{NUL}1722000726 ' len=12
at org.apache.commons.compress.archivers.tar.TarUtils.parseOctal(TarUtils.java:99)
at org.apache.commons.compress.archivers.tar.TarArchiveEntry.parseTarHeader(TarArchiveEntry.java:819)
at org.apache.commons.compress.archivers.tar.TarArchiveEntry.<init>(TarArchiveEntry.java:314)
at org.apache.commons.compress.archivers.tar.TarArchiveInputStream.getNextTarEntry(TarArchiveInputStream.java:199)
```
You are a professional Java test case writer, please create a test case named `workaroundForBrokenTimeHeader` for the issue `Compress-COMPRESS-181`, utilizing the provided issue report information and the following function signature.
```java
@Test
public void workaroundForBrokenTimeHeader() throws Exception {
```
| 49 | [
"org.apache.commons.compress.archivers.tar.TarUtils"
] | 848f11e5289c47b1a0e0e64694a1379ff22d06e6acee4370b10942472086371a | @Test
public void workaroundForBrokenTimeHeader() throws Exception | // You are a professional Java test case writer, please create a test case named `workaroundForBrokenTimeHeader` for the issue `Compress-COMPRESS-181`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Compress-COMPRESS-181
//
// ## Issue-Title:
// Tar files created by AIX native tar, and which contain symlinks, cannot be read by TarArchiveInputStream
//
// ## Issue-Description:
//
// A simple tar file created on AIX using the native (/usr/bin/tar tar utility) **and** which contains a symbolic link, cannot be loaded by TarArchiveInputStream:
//
//
//
//
// ```
// java.io.IOException: Error detected parsing the header
// at org.apache.commons.compress.archivers.tar.TarArchiveInputStream.getNextTarEntry(TarArchiveInputStream.java:201)
// at Extractor.extract(Extractor.java:13)
// at Extractor.main(Extractor.java:28)
// at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
// at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:39)
// at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:25)
// at java.lang.reflect.Method.invoke(Method.java:597)
// at org.apache.tools.ant.taskdefs.ExecuteJava.run(ExecuteJava.java:217)
// at org.apache.tools.ant.taskdefs.ExecuteJava.execute(ExecuteJava.java:152)
// at org.apache.tools.ant.taskdefs.Java.run(Java.java:771)
// at org.apache.tools.ant.taskdefs.Java.executeJava(Java.java:221)
// at org.apache.tools.ant.taskdefs.Java.executeJava(Java.java:135)
// at org.apache.tools.ant.taskdefs.Java.execute(Java.java:108)
// at org.apache.tools.ant.UnknownElement.execute(UnknownElement.java:291)
// at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
// at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:39)
// at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:25)
// at java.lang.reflect.Method.invoke(Method.java:597)
// at org.apache.tools.ant.dispatch.DispatchUtils.execute(DispatchUtils.java:106)
// at org.apache.tools.ant.Task.perform(Task.java:348)
// at org.apache.tools.ant.Target.execute(Target.java:390)
// at org.apache.tools.ant.Target.performTasks(Target.java:411)
// at org.apache.tools.ant.Project.executeSortedTargets(Project.java:1399)
// at org.apache.tools.ant.Project.executeTarget(Project.java:1368)
// at org.apache.tools.ant.helper.DefaultExecutor.executeTargets(DefaultExecutor.java:41)
// at org.apache.tools.ant.Project.executeTargets(Project.java:1251)
// at org.apache.tools.ant.Main.runBuild(Main.java:809)
// at org.apache.tools.ant.Main.startAnt(Main.java:217)
// at org.apache.tools.ant.launch.Launcher.run(Launcher.java:280)
// at org.apache.tools.ant.launch.Launcher.main(Launcher.java:109)
// Caused by: java.lang.IllegalArgumentException: Invalid byte 0 at offset 0 in '{NUL}1722000726 ' len=12
// at org.apache.commons.compress.archivers.tar.TarUtils.parseOctal(TarUtils.java:99)
// at org.apache.commons.compress.archivers.tar.TarArchiveEntry.parseTarHeader(TarArchiveEntry.java:819)
// at org.apache.commons.compress.archivers.tar.TarArchiveEntry.<init>(TarArchiveEntry.java:314)
// at org.apache.commons.compress.archivers.tar.TarArchiveInputStream.getNextTarEntry(TarArchiveInputStream.java:199)
| Compress | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package org.apache.commons.compress.archivers.tar;
import java.io.File;
import java.io.FileInputStream;
import java.io.StringReader;
import java.net.URI;
import java.net.URL;
import java.util.Date;
import java.util.Map;
import org.junit.Test;
import static org.junit.Assert.assertEquals;
import static org.junit.Assert.assertTrue;
public class TarArchiveInputStreamTest {
@Test
public void readSimplePaxHeader() throws Exception {
Map<String, String> headers = new TarArchiveInputStream(null)
.parsePaxHeaders(new StringReader("30 atime=1321711775.972059463\n"));
assertEquals(1, headers.size());
assertEquals("1321711775.972059463", headers.get("atime"));
}
@Test
public void readPaxHeaderWithEmbeddedNewline() throws Exception {
Map<String, String> headers = new TarArchiveInputStream(null)
.parsePaxHeaders(new StringReader("28 comment=line1\nline2\nand3\n"));
assertEquals(1, headers.size());
assertEquals("line1\nline2\nand3", headers.get("comment"));
}
@Test
public void workaroundForBrokenTimeHeader() throws Exception {
URL tar = getClass().getResource("/simple-aix-native-tar.tar");
TarArchiveInputStream in = null;
try {
in = new TarArchiveInputStream(new FileInputStream(new File(new URI(tar.toString()))));
TarArchiveEntry tae = in.getNextTarEntry();
tae = in.getNextTarEntry();
assertEquals("sample/link-to-txt-file.lnk", tae.getName());
assertEquals(new Date(0), tae.getLastModifiedDate());
assertTrue(tae.isSymbolicLink());
} finally {
if (in != null) {
in.close();
}
}
}
} |
||
public void testFailOnWritingStringNotFieldNameBytes() throws Exception {
_testFailOnWritingStringNotFieldName(F, false);
} | com.fasterxml.jackson.core.json.GeneratorFailTest::testFailOnWritingStringNotFieldNameBytes | src/test/java/com/fasterxml/jackson/core/json/GeneratorFailTest.java | 27 | src/test/java/com/fasterxml/jackson/core/json/GeneratorFailTest.java | testFailOnWritingStringNotFieldNameBytes | package com.fasterxml.jackson.core.json;
import java.io.ByteArrayOutputStream;
import java.io.OutputStreamWriter;
import com.fasterxml.jackson.core.JsonEncoding;
import com.fasterxml.jackson.core.JsonFactory;
import com.fasterxml.jackson.core.JsonGenerator;
import com.fasterxml.jackson.core.JsonProcessingException;
public class GeneratorFailTest
extends com.fasterxml.jackson.core.BaseTest
{
private final JsonFactory F = new JsonFactory();
// [core#167]: no error for writing field name twice
public void testDupFieldNameWrites() throws Exception
{
_testDupFieldNameWrites(F, false);
_testDupFieldNameWrites(F, true);
}
// [core#177]
// Also: should not try writing JSON String if field name expected
// (in future maybe take one as alias... but not yet)
public void testFailOnWritingStringNotFieldNameBytes() throws Exception {
_testFailOnWritingStringNotFieldName(F, false);
}
// [core#177]
public void testFailOnWritingStringNotFieldNameChars() throws Exception {
_testFailOnWritingStringNotFieldName(F, true);
}
/*
/**********************************************************
/* Internal methods
/**********************************************************
*/
private void _testDupFieldNameWrites(JsonFactory f, boolean useReader) throws Exception
{
JsonGenerator gen;
ByteArrayOutputStream bout = new ByteArrayOutputStream();
if (useReader) {
gen = f.createGenerator(new OutputStreamWriter(bout, "UTF-8"));
} else {
gen = f.createGenerator(bout, JsonEncoding.UTF8);
}
gen.writeStartObject();
gen.writeFieldName("a");
try {
gen.writeFieldName("b");
gen.flush();
String json = bout.toString("UTF-8");
fail("Should not have let two consecutive field name writes succeed: output = "+json);
} catch (JsonProcessingException e) {
verifyException(e, "can not write a field name, expecting a value");
}
gen.close();
}
private void _testFailOnWritingStringNotFieldName(JsonFactory f, boolean useReader) throws Exception
{
JsonGenerator gen;
ByteArrayOutputStream bout = new ByteArrayOutputStream();
if (useReader) {
gen = f.createGenerator(new OutputStreamWriter(bout, "UTF-8"));
} else {
gen = f.createGenerator(bout, JsonEncoding.UTF8);
}
gen.writeStartObject();
try {
gen.writeString("a");
gen.flush();
String json = bout.toString("UTF-8");
fail("Should not have let "+gen.getClass().getName()+".writeString() be used in place of 'writeFieldName()': output = "+json);
} catch (JsonProcessingException e) {
verifyException(e, "can not write a String");
}
gen.close();
}
} | // You are a professional Java test case writer, please create a test case named `testFailOnWritingStringNotFieldNameBytes` for the issue `JacksonCore-177`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonCore-177
//
// ## Issue-Title:
// Add a check so JsonGenerator.writeString() won't work if writeFieldName() expected.
//
// ## Issue-Description:
// Looks like calling `writeString()` (and perhaps other scalar write methods) results in writing invalid output, instead of throwing an exception. It should instead fail; in future we may want to consider allowing this as an alias, but at any rate it should not produce invalid output.
//
//
//
//
public void testFailOnWritingStringNotFieldNameBytes() throws Exception {
| 27 | // (in future maybe take one as alias... but not yet)
// Also: should not try writing JSON String if field name expected
// [core#177] | 7 | 25 | src/test/java/com/fasterxml/jackson/core/json/GeneratorFailTest.java | src/test/java | ```markdown
## Issue-ID: JacksonCore-177
## Issue-Title:
Add a check so JsonGenerator.writeString() won't work if writeFieldName() expected.
## Issue-Description:
Looks like calling `writeString()` (and perhaps other scalar write methods) results in writing invalid output, instead of throwing an exception. It should instead fail; in future we may want to consider allowing this as an alias, but at any rate it should not produce invalid output.
```
You are a professional Java test case writer, please create a test case named `testFailOnWritingStringNotFieldNameBytes` for the issue `JacksonCore-177`, utilizing the provided issue report information and the following function signature.
```java
public void testFailOnWritingStringNotFieldNameBytes() throws Exception {
```
| 25 | [
"com.fasterxml.jackson.core.json.JsonWriteContext"
] | 84cc9e8003666273b27236d509190eeee6cd418a93b72b0189fb598543e6c6db | public void testFailOnWritingStringNotFieldNameBytes() throws Exception | // You are a professional Java test case writer, please create a test case named `testFailOnWritingStringNotFieldNameBytes` for the issue `JacksonCore-177`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonCore-177
//
// ## Issue-Title:
// Add a check so JsonGenerator.writeString() won't work if writeFieldName() expected.
//
// ## Issue-Description:
// Looks like calling `writeString()` (and perhaps other scalar write methods) results in writing invalid output, instead of throwing an exception. It should instead fail; in future we may want to consider allowing this as an alias, but at any rate it should not produce invalid output.
//
//
//
//
| JacksonCore | package com.fasterxml.jackson.core.json;
import java.io.ByteArrayOutputStream;
import java.io.OutputStreamWriter;
import com.fasterxml.jackson.core.JsonEncoding;
import com.fasterxml.jackson.core.JsonFactory;
import com.fasterxml.jackson.core.JsonGenerator;
import com.fasterxml.jackson.core.JsonProcessingException;
public class GeneratorFailTest
extends com.fasterxml.jackson.core.BaseTest
{
private final JsonFactory F = new JsonFactory();
// [core#167]: no error for writing field name twice
public void testDupFieldNameWrites() throws Exception
{
_testDupFieldNameWrites(F, false);
_testDupFieldNameWrites(F, true);
}
// [core#177]
// Also: should not try writing JSON String if field name expected
// (in future maybe take one as alias... but not yet)
public void testFailOnWritingStringNotFieldNameBytes() throws Exception {
_testFailOnWritingStringNotFieldName(F, false);
}
// [core#177]
public void testFailOnWritingStringNotFieldNameChars() throws Exception {
_testFailOnWritingStringNotFieldName(F, true);
}
/*
/**********************************************************
/* Internal methods
/**********************************************************
*/
private void _testDupFieldNameWrites(JsonFactory f, boolean useReader) throws Exception
{
JsonGenerator gen;
ByteArrayOutputStream bout = new ByteArrayOutputStream();
if (useReader) {
gen = f.createGenerator(new OutputStreamWriter(bout, "UTF-8"));
} else {
gen = f.createGenerator(bout, JsonEncoding.UTF8);
}
gen.writeStartObject();
gen.writeFieldName("a");
try {
gen.writeFieldName("b");
gen.flush();
String json = bout.toString("UTF-8");
fail("Should not have let two consecutive field name writes succeed: output = "+json);
} catch (JsonProcessingException e) {
verifyException(e, "can not write a field name, expecting a value");
}
gen.close();
}
private void _testFailOnWritingStringNotFieldName(JsonFactory f, boolean useReader) throws Exception
{
JsonGenerator gen;
ByteArrayOutputStream bout = new ByteArrayOutputStream();
if (useReader) {
gen = f.createGenerator(new OutputStreamWriter(bout, "UTF-8"));
} else {
gen = f.createGenerator(bout, JsonEncoding.UTF8);
}
gen.writeStartObject();
try {
gen.writeString("a");
gen.flush();
String json = bout.toString("UTF-8");
fail("Should not have let "+gen.getClass().getName()+".writeString() be used in place of 'writeFieldName()': output = "+json);
} catch (JsonProcessingException e) {
verifyException(e, "can not write a String");
}
gen.close();
}
} |
|
@SuppressWarnings("unchecked")
public void testEqCondition4() throws Exception {
FlowScope blind = newScope();
testBinop(blind,
Token.EQ,
createVar(blind, "a", VOID_TYPE),
createVar(blind, "b", VOID_TYPE),
Sets.newHashSet(
new TypedName("a", VOID_TYPE),
new TypedName("b", VOID_TYPE)),
Sets.newHashSet(
new TypedName("a", NO_TYPE),
new TypedName("b", NO_TYPE)));
} | com.google.javascript.jscomp.SemanticReverseAbstractInterpreterTest::testEqCondition4 | test/com/google/javascript/jscomp/SemanticReverseAbstractInterpreterTest.java | 350 | test/com/google/javascript/jscomp/SemanticReverseAbstractInterpreterTest.java | testEqCondition4 | /*
* Copyright 2007 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import com.google.common.collect.Sets;
import com.google.javascript.rhino.Node;
import com.google.javascript.rhino.Token;
import com.google.javascript.rhino.jstype.JSType;
import java.util.Arrays;
import java.util.Collection;
public class SemanticReverseAbstractInterpreterTest
extends CompilerTypeTestCase {
private CodingConvention codingConvention = new GoogleCodingConvention();
private ReverseAbstractInterpreter interpreter;
private Scope functionScope;
@Override
protected void setUp() throws Exception {
super.setUp();
interpreter = new SemanticReverseAbstractInterpreter(
codingConvention, registry);
}
public FlowScope newScope() {
Scope globalScope = new Scope(new Node(Token.EMPTY), compiler);
functionScope = new Scope(globalScope, new Node(Token.EMPTY));
return LinkedFlowScope.createEntryLattice(functionScope);
}
/**
* Tests reverse interpretation of a NAME expression.
*/
public void testNameCondition() throws Exception {
FlowScope blind = newScope();
Node condition = createVar(blind, "a", createNullableType(STRING_TYPE));
// true outcome.
FlowScope informedTrue = interpreter.
getPreciserScopeKnowingConditionOutcome(condition, blind, true);
assertEquals(STRING_TYPE, getVarType(informedTrue, "a"));
// false outcome.
FlowScope informedFalse = interpreter.
getPreciserScopeKnowingConditionOutcome(condition, blind, false);
assertEquals(createNullableType(STRING_TYPE),
getVarType(informedFalse, "a"));
}
/**
* Tests reverse interpretation of a NOT(NAME) expression.
*/
public void testNegatedNameCondition() throws Exception {
FlowScope blind = newScope();
Node a = createVar(blind, "a", createNullableType(STRING_TYPE));
Node condition = new Node(Token.NOT);
condition.addChildToBack(a);
// true outcome.
FlowScope informedTrue = interpreter.
getPreciserScopeKnowingConditionOutcome(condition, blind, true);
assertEquals(createNullableType(STRING_TYPE),
getVarType(informedTrue, "a"));
// false outcome.
FlowScope informedFalse = interpreter.
getPreciserScopeKnowingConditionOutcome(condition, blind, false);
assertEquals(STRING_TYPE, getVarType(informedFalse, "a"));
}
/**
* Tests reverse interpretation of a ASSIGN expression.
*/
@SuppressWarnings("unchecked")
public void testAssignCondition1() throws Exception {
FlowScope blind = newScope();
testBinop(blind,
Token.ASSIGN,
createVar(blind, "a", createNullableType(OBJECT_TYPE)),
createVar(blind, "b", createNullableType(OBJECT_TYPE)),
Sets.newHashSet(
new TypedName("a", OBJECT_TYPE),
new TypedName("b", OBJECT_TYPE)),
Sets.newHashSet(
new TypedName("a", NULL_TYPE),
new TypedName("b", NULL_TYPE)));
}
/**
* Tests reverse interpretation of a SHEQ(NAME, NUMBER) expression.
*/
@SuppressWarnings("unchecked")
public void testSheqCondition1() throws Exception {
FlowScope blind = newScope();
testBinop(blind,
Token.SHEQ,
createVar(blind, "a", createUnionType(STRING_TYPE, NUMBER_TYPE)),
createNumber(56),
Sets.newHashSet(new TypedName("a", NUMBER_TYPE)),
Sets.newHashSet(new TypedName("a",
createUnionType(STRING_TYPE, NUMBER_TYPE))));
}
/**
* Tests reverse interpretation of a SHEQ(NUMBER, NAME) expression.
*/
@SuppressWarnings("unchecked")
public void testSheqCondition2() throws Exception {
FlowScope blind = newScope();
testBinop(blind,
Token.SHEQ,
createNumber(56),
createVar(blind, "a", createUnionType(STRING_TYPE, NUMBER_TYPE)),
Sets.newHashSet(new TypedName("a", NUMBER_TYPE)),
Sets.newHashSet(new TypedName("a",
createUnionType(STRING_TYPE, NUMBER_TYPE))));
}
/**
* Tests reverse interpretation of a SHEQ(NAME, NAME) expression.
*/
@SuppressWarnings("unchecked")
public void testSheqCondition3() throws Exception {
FlowScope blind = newScope();
testBinop(blind,
Token.SHEQ,
createVar(blind, "b", createUnionType(STRING_TYPE, BOOLEAN_TYPE)),
createVar(blind, "a", createUnionType(STRING_TYPE, NUMBER_TYPE)),
Sets.newHashSet(new TypedName("a", STRING_TYPE),
new TypedName("b", STRING_TYPE)),
Sets.newHashSet(new TypedName("a",
createUnionType(STRING_TYPE, NUMBER_TYPE)),
new TypedName("b",
createUnionType(STRING_TYPE, BOOLEAN_TYPE))));
}
@SuppressWarnings("unchecked")
public void testSheqCondition4() throws Exception {
FlowScope blind = newScope();
testBinop(blind,
Token.SHEQ,
createVar(blind, "a", createUnionType(STRING_TYPE, VOID_TYPE)),
createVar(blind, "b", createUnionType(VOID_TYPE)),
Sets.newHashSet(new TypedName("a", VOID_TYPE),
new TypedName("b", VOID_TYPE)),
Sets.newHashSet(new TypedName("a", STRING_TYPE),
new TypedName("b", VOID_TYPE)));
}
@SuppressWarnings("unchecked")
public void testSheqCondition5() throws Exception {
FlowScope blind = newScope();
testBinop(blind,
Token.SHEQ,
createVar(blind, "a", createUnionType(NULL_TYPE, VOID_TYPE)),
createVar(blind, "b", createUnionType(VOID_TYPE)),
Sets.newHashSet(new TypedName("a", VOID_TYPE),
new TypedName("b", VOID_TYPE)),
Sets.newHashSet(new TypedName("a", NULL_TYPE),
new TypedName("b", VOID_TYPE)));
}
@SuppressWarnings("unchecked")
public void testSheqCondition6() throws Exception {
FlowScope blind = newScope();
testBinop(blind,
Token.SHEQ,
createVar(blind, "a", createUnionType(STRING_TYPE, VOID_TYPE)),
createVar(blind, "b", createUnionType(NUMBER_TYPE, VOID_TYPE)),
Sets.newHashSet(
new TypedName("a", VOID_TYPE),
new TypedName("b", VOID_TYPE)),
Sets.newHashSet(
new TypedName("a",
createUnionType(STRING_TYPE, VOID_TYPE)),
new TypedName("b",
createUnionType(NUMBER_TYPE, VOID_TYPE))));
}
/**
* Tests reverse interpretation of a SHNE(NAME, NUMBER) expression.
*/
@SuppressWarnings("unchecked")
public void testShneCondition1() throws Exception {
FlowScope blind = newScope();
testBinop(blind,
Token.SHNE,
createVar(blind, "a", createUnionType(STRING_TYPE, NUMBER_TYPE)),
createNumber(56),
Sets.newHashSet(new TypedName("a",
createUnionType(STRING_TYPE, NUMBER_TYPE))),
Sets.newHashSet(new TypedName("a", NUMBER_TYPE)));
}
/**
* Tests reverse interpretation of a SHNE(NUMBER, NAME) expression.
*/
@SuppressWarnings("unchecked")
public void testShneCondition2() throws Exception {
FlowScope blind = newScope();
testBinop(blind,
Token.SHNE,
createNumber(56),
createVar(blind, "a", createUnionType(STRING_TYPE, NUMBER_TYPE)),
Sets.newHashSet(new TypedName("a",
createUnionType(STRING_TYPE, NUMBER_TYPE))),
Sets.newHashSet(new TypedName("a", NUMBER_TYPE)));
}
/**
* Tests reverse interpretation of a SHNE(NAME, NAME) expression.
*/
@SuppressWarnings("unchecked")
public void testShneCondition3() throws Exception {
FlowScope blind = newScope();
testBinop(blind,
Token.SHNE,
createVar(blind, "b", createUnionType(STRING_TYPE, BOOLEAN_TYPE)),
createVar(blind, "a", createUnionType(STRING_TYPE, NUMBER_TYPE)),
Sets.newHashSet(new TypedName("a",
createUnionType(STRING_TYPE, NUMBER_TYPE)),
new TypedName("b",
createUnionType(STRING_TYPE, BOOLEAN_TYPE))),
Sets.newHashSet(new TypedName("a", STRING_TYPE),
new TypedName("b", STRING_TYPE)));
}
@SuppressWarnings("unchecked")
public void testShneCondition4() throws Exception {
FlowScope blind = newScope();
testBinop(blind,
Token.SHNE,
createVar(blind, "a", createUnionType(STRING_TYPE, VOID_TYPE)),
createVar(blind, "b", createUnionType(VOID_TYPE)),
Sets.newHashSet(new TypedName("a", STRING_TYPE),
new TypedName("b", VOID_TYPE)),
Sets.newHashSet(new TypedName("a", VOID_TYPE),
new TypedName("b", VOID_TYPE)));
}
@SuppressWarnings("unchecked")
public void testShneCondition5() throws Exception {
FlowScope blind = newScope();
testBinop(blind,
Token.SHNE,
createVar(blind, "a", createUnionType(NULL_TYPE, VOID_TYPE)),
createVar(blind, "b", createUnionType(NULL_TYPE)),
Sets.newHashSet(new TypedName("a", VOID_TYPE),
new TypedName("b", NULL_TYPE)),
Sets.newHashSet(new TypedName("a", NULL_TYPE),
new TypedName("b", NULL_TYPE)));
}
@SuppressWarnings("unchecked")
public void testShneCondition6() throws Exception {
FlowScope blind = newScope();
testBinop(blind,
Token.SHNE,
createVar(blind, "a", createUnionType(STRING_TYPE, VOID_TYPE)),
createVar(blind, "b", createUnionType(NUMBER_TYPE, VOID_TYPE)),
Sets.newHashSet(
new TypedName("a",
createUnionType(STRING_TYPE, VOID_TYPE)),
new TypedName("b",
createUnionType(NUMBER_TYPE, VOID_TYPE))),
Sets.newHashSet(
new TypedName("a", VOID_TYPE),
new TypedName("b", VOID_TYPE)));
}
/**
* Tests reverse interpretation of a EQ(NAME, NULL) expression.
*/
@SuppressWarnings("unchecked")
public void testEqCondition1() throws Exception {
FlowScope blind = newScope();
testBinop(blind,
Token.EQ,
createVar(blind, "a", createUnionType(BOOLEAN_TYPE, VOID_TYPE)),
createNull(),
Sets.newHashSet(new TypedName("a", VOID_TYPE)),
Sets.newHashSet(new TypedName("a", BOOLEAN_TYPE)));
}
/**
* Tests reverse interpretation of a NE(NULL, NAME) expression.
*/
@SuppressWarnings("unchecked")
public void testEqCondition2() throws Exception {
FlowScope blind = newScope();
testBinop(blind,
Token.NE,
createNull(),
createVar(blind, "a", createUnionType(BOOLEAN_TYPE, VOID_TYPE)),
Sets.newHashSet(new TypedName("a", BOOLEAN_TYPE)),
Sets.newHashSet(new TypedName("a", VOID_TYPE)));
}
/**
* Tests reverse interpretation of a EQ(NAME, NULL) expression.
*/
@SuppressWarnings("unchecked")
public void testEqCondition3() throws Exception {
FlowScope blind = newScope();
// (number,undefined,null)
JSType nullableOptionalNumber =
createUnionType(NULL_TYPE, VOID_TYPE, NUMBER_TYPE);
// (null,undefined)
JSType nullUndefined =
createUnionType(VOID_TYPE, NULL_TYPE);
testBinop(blind,
Token.EQ,
createVar(blind, "a", nullableOptionalNumber),
createNull(),
Sets.newHashSet(new TypedName("a", nullUndefined)),
Sets.newHashSet(new TypedName("a", NUMBER_TYPE)));
}
/**
* Tests reverse interpretation of two undefineds.
*/
@SuppressWarnings("unchecked")
public void testEqCondition4() throws Exception {
FlowScope blind = newScope();
testBinop(blind,
Token.EQ,
createVar(blind, "a", VOID_TYPE),
createVar(blind, "b", VOID_TYPE),
Sets.newHashSet(
new TypedName("a", VOID_TYPE),
new TypedName("b", VOID_TYPE)),
Sets.newHashSet(
new TypedName("a", NO_TYPE),
new TypedName("b", NO_TYPE)));
}
/**
* Tests reverse interpretation of a COMPARE(NAME, NUMBER) expression,
* where COMPARE can be LE, LT, GE or GT.
*/
@SuppressWarnings("unchecked")
public void testInequalitiesCondition1() {
for (int op : Arrays.asList(Token.LT, Token.GT, Token.LE, Token.GE)) {
FlowScope blind = newScope();
testBinop(blind,
op,
createVar(blind, "a", createUnionType(STRING_TYPE, VOID_TYPE)),
createNumber(8),
Sets.newHashSet(
new TypedName("a", STRING_TYPE)),
Sets.newHashSet(new TypedName("a",
createUnionType(STRING_TYPE, VOID_TYPE))));
}
}
/**
* Tests reverse interpretation of a COMPARE(NAME, NAME) expression,
* where COMPARE can be LE, LT, GE or GT.
*/
@SuppressWarnings("unchecked")
public void testInequalitiesCondition2() {
for (int op : Arrays.asList(Token.LT, Token.GT, Token.LE, Token.GE)) {
FlowScope blind = newScope();
testBinop(blind,
op,
createVar(blind, "a",
createUnionType(STRING_TYPE, NUMBER_TYPE, VOID_TYPE)),
createVar(blind, "b",
createUnionType(NUMBER_TYPE, NULL_TYPE)),
Sets.newHashSet(
new TypedName("a",
createUnionType(STRING_TYPE, NUMBER_TYPE)),
new TypedName("b",
createUnionType(NUMBER_TYPE, NULL_TYPE))),
Sets.newHashSet(
new TypedName("a",
createUnionType(STRING_TYPE, NUMBER_TYPE, VOID_TYPE)),
new TypedName("b",
createUnionType(NUMBER_TYPE, NULL_TYPE))));
}
}
/**
* Tests reverse interpretation of a COMPARE(NUMBER-untyped, NAME) expression,
* where COMPARE can be LE, LT, GE or GT.
*/
@SuppressWarnings("unchecked")
public void testInequalitiesCondition3() {
for (int op : Arrays.asList(Token.LT, Token.GT, Token.LE, Token.GE)) {
FlowScope blind = newScope();
testBinop(blind,
op,
createUntypedNumber(8),
createVar(blind, "a", createUnionType(STRING_TYPE, VOID_TYPE)),
Sets.newHashSet(
new TypedName("a", STRING_TYPE)),
Sets.newHashSet(new TypedName("a",
createUnionType(STRING_TYPE, VOID_TYPE))));
}
}
@SuppressWarnings("unchecked")
public void testAnd() {
FlowScope blind = newScope();
testBinop(blind,
Token.AND,
createVar(blind, "b", createUnionType(STRING_TYPE, NULL_TYPE)),
createVar(blind, "a", createUnionType(NUMBER_TYPE, VOID_TYPE)),
Sets.newHashSet(new TypedName("a", NUMBER_TYPE),
new TypedName("b", STRING_TYPE)),
Sets.newHashSet(new TypedName("a",
createUnionType(NUMBER_TYPE, VOID_TYPE)),
new TypedName("b",
createUnionType(STRING_TYPE, NULL_TYPE))));
}
@SuppressWarnings("unchecked")
public void testTypeof1() {
FlowScope blind = newScope();
testBinop(blind,
Token.EQ,
new Node(Token.TYPEOF, createVar(blind, "a", OBJECT_TYPE)),
Node.newString("function"),
Sets.newHashSet(
new TypedName("a", U2U_CONSTRUCTOR_TYPE)),
Sets.newHashSet(
new TypedName("a", OBJECT_TYPE)));
}
@SuppressWarnings("unchecked")
public void testTypeof2() {
FlowScope blind = newScope();
testBinop(blind,
Token.EQ,
new Node(Token.TYPEOF, createVar(blind, "a", ALL_TYPE)),
Node.newString("function"),
Sets.newHashSet(
new TypedName("a", U2U_CONSTRUCTOR_TYPE)),
Sets.newHashSet(
new TypedName("a", ALL_TYPE)));
}
@SuppressWarnings("unchecked")
public void testInstanceOf() {
FlowScope blind = newScope();
testBinop(blind,
Token.INSTANCEOF,
createVar(blind, "x", UNKNOWN_TYPE),
createVar(blind, "s", STRING_OBJECT_FUNCTION_TYPE),
Sets.newHashSet(
new TypedName("x", STRING_OBJECT_TYPE),
new TypedName("s", STRING_OBJECT_FUNCTION_TYPE)),
Sets.newHashSet(
new TypedName("s", STRING_OBJECT_FUNCTION_TYPE)));
}
@SuppressWarnings("unchecked")
public void testInstanceOf2() {
FlowScope blind = newScope();
testBinop(blind,
Token.INSTANCEOF,
createVar(blind, "x",
createUnionType(STRING_OBJECT_TYPE, NUMBER_OBJECT_TYPE)),
createVar(blind, "s", STRING_OBJECT_FUNCTION_TYPE),
Sets.newHashSet(
new TypedName("x", STRING_OBJECT_TYPE),
new TypedName("s", STRING_OBJECT_FUNCTION_TYPE)),
Sets.newHashSet(
new TypedName("x", NUMBER_OBJECT_TYPE),
new TypedName("s", STRING_OBJECT_FUNCTION_TYPE)));
}
@SuppressWarnings("unchecked")
public void testInstanceOf3() {
FlowScope blind = newScope();
testBinop(blind,
Token.INSTANCEOF,
createVar(blind, "x", OBJECT_TYPE),
createVar(blind, "s", STRING_OBJECT_FUNCTION_TYPE),
Sets.newHashSet(
new TypedName("x", STRING_OBJECT_TYPE),
new TypedName("s", STRING_OBJECT_FUNCTION_TYPE)),
Sets.newHashSet(
new TypedName("x", OBJECT_TYPE),
new TypedName("s", STRING_OBJECT_FUNCTION_TYPE)));
}
@SuppressWarnings("unchecked")
public void testInstanceOf4() {
FlowScope blind = newScope();
testBinop(blind,
Token.INSTANCEOF,
createVar(blind, "x", ALL_TYPE),
createVar(blind, "s", STRING_OBJECT_FUNCTION_TYPE),
Sets.newHashSet(
new TypedName("x", STRING_OBJECT_TYPE),
new TypedName("s", STRING_OBJECT_FUNCTION_TYPE)),
Sets.newHashSet(
new TypedName("s", STRING_OBJECT_FUNCTION_TYPE)));
}
private void testBinop(FlowScope blind, int binop, Node left, Node right,
Collection<TypedName> trueOutcome,
Collection<TypedName> falseOutcome) {
Node condition = new Node(binop);
condition.addChildToBack(left);
condition.addChildToBack(right);
// true outcome.
FlowScope informedTrue = interpreter.
getPreciserScopeKnowingConditionOutcome(condition, blind, true);
for (TypedName p : trueOutcome) {
assertEquals(p.name, p.type, getVarType(informedTrue, p.name));
}
// false outcome.
FlowScope informedFalse = interpreter.
getPreciserScopeKnowingConditionOutcome(condition, blind, false);
for (TypedName p : falseOutcome) {
assertEquals(p.type, getVarType(informedFalse, p.name));
}
}
private Node createNull() {
Node n = new Node(Token.NULL);
n.setJSType(NULL_TYPE);
return n;
}
private Node createNumber(int n) {
Node number = createUntypedNumber(n);
number.setJSType(NUMBER_TYPE);
return number;
}
private Node createUntypedNumber(int n) {
return Node.newNumber(n);
}
private JSType getVarType(FlowScope scope, String name) {
return scope.getSlot(name).getType();
}
private Node createVar(FlowScope scope, String name, JSType type) {
Node n = Node.newString(Token.NAME, name);
functionScope.declare(name, n, null, null);
((LinkedFlowScope) scope).inferSlotType(name, type);
n.setJSType(type);
return n;
}
private static class TypedName {
private final String name;
private final JSType type;
private TypedName(String name, JSType type) {
this.name = name;
this.type = type;
}
}
} | // You are a professional Java test case writer, please create a test case named `testEqCondition4` for the issue `Closure-172`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-172
//
// ## Issue-Title:
// bad type inference for != undefined
//
// ## Issue-Description:
// **What steps will reproduce the problem?**
//
// // ==ClosureCompiler==
// // @compilation\_level ADVANCED\_OPTIMIZATIONS
// // @output\_file\_name default.js
// // ==/ClosureCompiler==
//
// /\*\* @param {string} x \*/
// function g(x) {}
//
// /\*\* @param {undefined} x \*/
// function f(x) {
// if (x != undefined) { g(x); }
// }
//
// **What is the expected output? What do you see instead?**
//
// JSC\_DETERMINISTIC\_TEST: condition always evaluates to false
// left : undefined
// right: undefined at line 6 character 6
// if (x != undefined) { g(x); }
// ^
// JSC\_TYPE\_MISMATCH: actual parameter 1 of g does not match formal parameter
// found : undefined
// required: string at line 6 character 24
// if (x != undefined) { g(x); }
// ^
//
// the second warning is bogus.
//
//
@SuppressWarnings("unchecked")
public void testEqCondition4() throws Exception {
| 350 | /**
* Tests reverse interpretation of two undefineds.
*/ | 146 | 337 | test/com/google/javascript/jscomp/SemanticReverseAbstractInterpreterTest.java | test | ```markdown
## Issue-ID: Closure-172
## Issue-Title:
bad type inference for != undefined
## Issue-Description:
**What steps will reproduce the problem?**
// ==ClosureCompiler==
// @compilation\_level ADVANCED\_OPTIMIZATIONS
// @output\_file\_name default.js
// ==/ClosureCompiler==
/\*\* @param {string} x \*/
function g(x) {}
/\*\* @param {undefined} x \*/
function f(x) {
if (x != undefined) { g(x); }
}
**What is the expected output? What do you see instead?**
JSC\_DETERMINISTIC\_TEST: condition always evaluates to false
left : undefined
right: undefined at line 6 character 6
if (x != undefined) { g(x); }
^
JSC\_TYPE\_MISMATCH: actual parameter 1 of g does not match formal parameter
found : undefined
required: string at line 6 character 24
if (x != undefined) { g(x); }
^
the second warning is bogus.
```
You are a professional Java test case writer, please create a test case named `testEqCondition4` for the issue `Closure-172`, utilizing the provided issue report information and the following function signature.
```java
@SuppressWarnings("unchecked")
public void testEqCondition4() throws Exception {
```
| 337 | [
"com.google.javascript.rhino.jstype.JSType"
] | 8589e62ad5fa4b34d2e3fa697eda356ab8f0a7afb75704b1c484727edbd1ab2a | @SuppressWarnings("unchecked")
public void testEqCondition4() throws Exception | // You are a professional Java test case writer, please create a test case named `testEqCondition4` for the issue `Closure-172`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-172
//
// ## Issue-Title:
// bad type inference for != undefined
//
// ## Issue-Description:
// **What steps will reproduce the problem?**
//
// // ==ClosureCompiler==
// // @compilation\_level ADVANCED\_OPTIMIZATIONS
// // @output\_file\_name default.js
// // ==/ClosureCompiler==
//
// /\*\* @param {string} x \*/
// function g(x) {}
//
// /\*\* @param {undefined} x \*/
// function f(x) {
// if (x != undefined) { g(x); }
// }
//
// **What is the expected output? What do you see instead?**
//
// JSC\_DETERMINISTIC\_TEST: condition always evaluates to false
// left : undefined
// right: undefined at line 6 character 6
// if (x != undefined) { g(x); }
// ^
// JSC\_TYPE\_MISMATCH: actual parameter 1 of g does not match formal parameter
// found : undefined
// required: string at line 6 character 24
// if (x != undefined) { g(x); }
// ^
//
// the second warning is bogus.
//
//
| Closure | /*
* Copyright 2007 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import com.google.common.collect.Sets;
import com.google.javascript.rhino.Node;
import com.google.javascript.rhino.Token;
import com.google.javascript.rhino.jstype.JSType;
import java.util.Arrays;
import java.util.Collection;
public class SemanticReverseAbstractInterpreterTest
extends CompilerTypeTestCase {
private CodingConvention codingConvention = new GoogleCodingConvention();
private ReverseAbstractInterpreter interpreter;
private Scope functionScope;
@Override
protected void setUp() throws Exception {
super.setUp();
interpreter = new SemanticReverseAbstractInterpreter(
codingConvention, registry);
}
public FlowScope newScope() {
Scope globalScope = new Scope(new Node(Token.EMPTY), compiler);
functionScope = new Scope(globalScope, new Node(Token.EMPTY));
return LinkedFlowScope.createEntryLattice(functionScope);
}
/**
* Tests reverse interpretation of a NAME expression.
*/
public void testNameCondition() throws Exception {
FlowScope blind = newScope();
Node condition = createVar(blind, "a", createNullableType(STRING_TYPE));
// true outcome.
FlowScope informedTrue = interpreter.
getPreciserScopeKnowingConditionOutcome(condition, blind, true);
assertEquals(STRING_TYPE, getVarType(informedTrue, "a"));
// false outcome.
FlowScope informedFalse = interpreter.
getPreciserScopeKnowingConditionOutcome(condition, blind, false);
assertEquals(createNullableType(STRING_TYPE),
getVarType(informedFalse, "a"));
}
/**
* Tests reverse interpretation of a NOT(NAME) expression.
*/
public void testNegatedNameCondition() throws Exception {
FlowScope blind = newScope();
Node a = createVar(blind, "a", createNullableType(STRING_TYPE));
Node condition = new Node(Token.NOT);
condition.addChildToBack(a);
// true outcome.
FlowScope informedTrue = interpreter.
getPreciserScopeKnowingConditionOutcome(condition, blind, true);
assertEquals(createNullableType(STRING_TYPE),
getVarType(informedTrue, "a"));
// false outcome.
FlowScope informedFalse = interpreter.
getPreciserScopeKnowingConditionOutcome(condition, blind, false);
assertEquals(STRING_TYPE, getVarType(informedFalse, "a"));
}
/**
* Tests reverse interpretation of a ASSIGN expression.
*/
@SuppressWarnings("unchecked")
public void testAssignCondition1() throws Exception {
FlowScope blind = newScope();
testBinop(blind,
Token.ASSIGN,
createVar(blind, "a", createNullableType(OBJECT_TYPE)),
createVar(blind, "b", createNullableType(OBJECT_TYPE)),
Sets.newHashSet(
new TypedName("a", OBJECT_TYPE),
new TypedName("b", OBJECT_TYPE)),
Sets.newHashSet(
new TypedName("a", NULL_TYPE),
new TypedName("b", NULL_TYPE)));
}
/**
* Tests reverse interpretation of a SHEQ(NAME, NUMBER) expression.
*/
@SuppressWarnings("unchecked")
public void testSheqCondition1() throws Exception {
FlowScope blind = newScope();
testBinop(blind,
Token.SHEQ,
createVar(blind, "a", createUnionType(STRING_TYPE, NUMBER_TYPE)),
createNumber(56),
Sets.newHashSet(new TypedName("a", NUMBER_TYPE)),
Sets.newHashSet(new TypedName("a",
createUnionType(STRING_TYPE, NUMBER_TYPE))));
}
/**
* Tests reverse interpretation of a SHEQ(NUMBER, NAME) expression.
*/
@SuppressWarnings("unchecked")
public void testSheqCondition2() throws Exception {
FlowScope blind = newScope();
testBinop(blind,
Token.SHEQ,
createNumber(56),
createVar(blind, "a", createUnionType(STRING_TYPE, NUMBER_TYPE)),
Sets.newHashSet(new TypedName("a", NUMBER_TYPE)),
Sets.newHashSet(new TypedName("a",
createUnionType(STRING_TYPE, NUMBER_TYPE))));
}
/**
* Tests reverse interpretation of a SHEQ(NAME, NAME) expression.
*/
@SuppressWarnings("unchecked")
public void testSheqCondition3() throws Exception {
FlowScope blind = newScope();
testBinop(blind,
Token.SHEQ,
createVar(blind, "b", createUnionType(STRING_TYPE, BOOLEAN_TYPE)),
createVar(blind, "a", createUnionType(STRING_TYPE, NUMBER_TYPE)),
Sets.newHashSet(new TypedName("a", STRING_TYPE),
new TypedName("b", STRING_TYPE)),
Sets.newHashSet(new TypedName("a",
createUnionType(STRING_TYPE, NUMBER_TYPE)),
new TypedName("b",
createUnionType(STRING_TYPE, BOOLEAN_TYPE))));
}
@SuppressWarnings("unchecked")
public void testSheqCondition4() throws Exception {
FlowScope blind = newScope();
testBinop(blind,
Token.SHEQ,
createVar(blind, "a", createUnionType(STRING_TYPE, VOID_TYPE)),
createVar(blind, "b", createUnionType(VOID_TYPE)),
Sets.newHashSet(new TypedName("a", VOID_TYPE),
new TypedName("b", VOID_TYPE)),
Sets.newHashSet(new TypedName("a", STRING_TYPE),
new TypedName("b", VOID_TYPE)));
}
@SuppressWarnings("unchecked")
public void testSheqCondition5() throws Exception {
FlowScope blind = newScope();
testBinop(blind,
Token.SHEQ,
createVar(blind, "a", createUnionType(NULL_TYPE, VOID_TYPE)),
createVar(blind, "b", createUnionType(VOID_TYPE)),
Sets.newHashSet(new TypedName("a", VOID_TYPE),
new TypedName("b", VOID_TYPE)),
Sets.newHashSet(new TypedName("a", NULL_TYPE),
new TypedName("b", VOID_TYPE)));
}
@SuppressWarnings("unchecked")
public void testSheqCondition6() throws Exception {
FlowScope blind = newScope();
testBinop(blind,
Token.SHEQ,
createVar(blind, "a", createUnionType(STRING_TYPE, VOID_TYPE)),
createVar(blind, "b", createUnionType(NUMBER_TYPE, VOID_TYPE)),
Sets.newHashSet(
new TypedName("a", VOID_TYPE),
new TypedName("b", VOID_TYPE)),
Sets.newHashSet(
new TypedName("a",
createUnionType(STRING_TYPE, VOID_TYPE)),
new TypedName("b",
createUnionType(NUMBER_TYPE, VOID_TYPE))));
}
/**
* Tests reverse interpretation of a SHNE(NAME, NUMBER) expression.
*/
@SuppressWarnings("unchecked")
public void testShneCondition1() throws Exception {
FlowScope blind = newScope();
testBinop(blind,
Token.SHNE,
createVar(blind, "a", createUnionType(STRING_TYPE, NUMBER_TYPE)),
createNumber(56),
Sets.newHashSet(new TypedName("a",
createUnionType(STRING_TYPE, NUMBER_TYPE))),
Sets.newHashSet(new TypedName("a", NUMBER_TYPE)));
}
/**
* Tests reverse interpretation of a SHNE(NUMBER, NAME) expression.
*/
@SuppressWarnings("unchecked")
public void testShneCondition2() throws Exception {
FlowScope blind = newScope();
testBinop(blind,
Token.SHNE,
createNumber(56),
createVar(blind, "a", createUnionType(STRING_TYPE, NUMBER_TYPE)),
Sets.newHashSet(new TypedName("a",
createUnionType(STRING_TYPE, NUMBER_TYPE))),
Sets.newHashSet(new TypedName("a", NUMBER_TYPE)));
}
/**
* Tests reverse interpretation of a SHNE(NAME, NAME) expression.
*/
@SuppressWarnings("unchecked")
public void testShneCondition3() throws Exception {
FlowScope blind = newScope();
testBinop(blind,
Token.SHNE,
createVar(blind, "b", createUnionType(STRING_TYPE, BOOLEAN_TYPE)),
createVar(blind, "a", createUnionType(STRING_TYPE, NUMBER_TYPE)),
Sets.newHashSet(new TypedName("a",
createUnionType(STRING_TYPE, NUMBER_TYPE)),
new TypedName("b",
createUnionType(STRING_TYPE, BOOLEAN_TYPE))),
Sets.newHashSet(new TypedName("a", STRING_TYPE),
new TypedName("b", STRING_TYPE)));
}
@SuppressWarnings("unchecked")
public void testShneCondition4() throws Exception {
FlowScope blind = newScope();
testBinop(blind,
Token.SHNE,
createVar(blind, "a", createUnionType(STRING_TYPE, VOID_TYPE)),
createVar(blind, "b", createUnionType(VOID_TYPE)),
Sets.newHashSet(new TypedName("a", STRING_TYPE),
new TypedName("b", VOID_TYPE)),
Sets.newHashSet(new TypedName("a", VOID_TYPE),
new TypedName("b", VOID_TYPE)));
}
@SuppressWarnings("unchecked")
public void testShneCondition5() throws Exception {
FlowScope blind = newScope();
testBinop(blind,
Token.SHNE,
createVar(blind, "a", createUnionType(NULL_TYPE, VOID_TYPE)),
createVar(blind, "b", createUnionType(NULL_TYPE)),
Sets.newHashSet(new TypedName("a", VOID_TYPE),
new TypedName("b", NULL_TYPE)),
Sets.newHashSet(new TypedName("a", NULL_TYPE),
new TypedName("b", NULL_TYPE)));
}
@SuppressWarnings("unchecked")
public void testShneCondition6() throws Exception {
FlowScope blind = newScope();
testBinop(blind,
Token.SHNE,
createVar(blind, "a", createUnionType(STRING_TYPE, VOID_TYPE)),
createVar(blind, "b", createUnionType(NUMBER_TYPE, VOID_TYPE)),
Sets.newHashSet(
new TypedName("a",
createUnionType(STRING_TYPE, VOID_TYPE)),
new TypedName("b",
createUnionType(NUMBER_TYPE, VOID_TYPE))),
Sets.newHashSet(
new TypedName("a", VOID_TYPE),
new TypedName("b", VOID_TYPE)));
}
/**
* Tests reverse interpretation of a EQ(NAME, NULL) expression.
*/
@SuppressWarnings("unchecked")
public void testEqCondition1() throws Exception {
FlowScope blind = newScope();
testBinop(blind,
Token.EQ,
createVar(blind, "a", createUnionType(BOOLEAN_TYPE, VOID_TYPE)),
createNull(),
Sets.newHashSet(new TypedName("a", VOID_TYPE)),
Sets.newHashSet(new TypedName("a", BOOLEAN_TYPE)));
}
/**
* Tests reverse interpretation of a NE(NULL, NAME) expression.
*/
@SuppressWarnings("unchecked")
public void testEqCondition2() throws Exception {
FlowScope blind = newScope();
testBinop(blind,
Token.NE,
createNull(),
createVar(blind, "a", createUnionType(BOOLEAN_TYPE, VOID_TYPE)),
Sets.newHashSet(new TypedName("a", BOOLEAN_TYPE)),
Sets.newHashSet(new TypedName("a", VOID_TYPE)));
}
/**
* Tests reverse interpretation of a EQ(NAME, NULL) expression.
*/
@SuppressWarnings("unchecked")
public void testEqCondition3() throws Exception {
FlowScope blind = newScope();
// (number,undefined,null)
JSType nullableOptionalNumber =
createUnionType(NULL_TYPE, VOID_TYPE, NUMBER_TYPE);
// (null,undefined)
JSType nullUndefined =
createUnionType(VOID_TYPE, NULL_TYPE);
testBinop(blind,
Token.EQ,
createVar(blind, "a", nullableOptionalNumber),
createNull(),
Sets.newHashSet(new TypedName("a", nullUndefined)),
Sets.newHashSet(new TypedName("a", NUMBER_TYPE)));
}
/**
* Tests reverse interpretation of two undefineds.
*/
@SuppressWarnings("unchecked")
public void testEqCondition4() throws Exception {
FlowScope blind = newScope();
testBinop(blind,
Token.EQ,
createVar(blind, "a", VOID_TYPE),
createVar(blind, "b", VOID_TYPE),
Sets.newHashSet(
new TypedName("a", VOID_TYPE),
new TypedName("b", VOID_TYPE)),
Sets.newHashSet(
new TypedName("a", NO_TYPE),
new TypedName("b", NO_TYPE)));
}
/**
* Tests reverse interpretation of a COMPARE(NAME, NUMBER) expression,
* where COMPARE can be LE, LT, GE or GT.
*/
@SuppressWarnings("unchecked")
public void testInequalitiesCondition1() {
for (int op : Arrays.asList(Token.LT, Token.GT, Token.LE, Token.GE)) {
FlowScope blind = newScope();
testBinop(blind,
op,
createVar(blind, "a", createUnionType(STRING_TYPE, VOID_TYPE)),
createNumber(8),
Sets.newHashSet(
new TypedName("a", STRING_TYPE)),
Sets.newHashSet(new TypedName("a",
createUnionType(STRING_TYPE, VOID_TYPE))));
}
}
/**
* Tests reverse interpretation of a COMPARE(NAME, NAME) expression,
* where COMPARE can be LE, LT, GE or GT.
*/
@SuppressWarnings("unchecked")
public void testInequalitiesCondition2() {
for (int op : Arrays.asList(Token.LT, Token.GT, Token.LE, Token.GE)) {
FlowScope blind = newScope();
testBinop(blind,
op,
createVar(blind, "a",
createUnionType(STRING_TYPE, NUMBER_TYPE, VOID_TYPE)),
createVar(blind, "b",
createUnionType(NUMBER_TYPE, NULL_TYPE)),
Sets.newHashSet(
new TypedName("a",
createUnionType(STRING_TYPE, NUMBER_TYPE)),
new TypedName("b",
createUnionType(NUMBER_TYPE, NULL_TYPE))),
Sets.newHashSet(
new TypedName("a",
createUnionType(STRING_TYPE, NUMBER_TYPE, VOID_TYPE)),
new TypedName("b",
createUnionType(NUMBER_TYPE, NULL_TYPE))));
}
}
/**
* Tests reverse interpretation of a COMPARE(NUMBER-untyped, NAME) expression,
* where COMPARE can be LE, LT, GE or GT.
*/
@SuppressWarnings("unchecked")
public void testInequalitiesCondition3() {
for (int op : Arrays.asList(Token.LT, Token.GT, Token.LE, Token.GE)) {
FlowScope blind = newScope();
testBinop(blind,
op,
createUntypedNumber(8),
createVar(blind, "a", createUnionType(STRING_TYPE, VOID_TYPE)),
Sets.newHashSet(
new TypedName("a", STRING_TYPE)),
Sets.newHashSet(new TypedName("a",
createUnionType(STRING_TYPE, VOID_TYPE))));
}
}
@SuppressWarnings("unchecked")
public void testAnd() {
FlowScope blind = newScope();
testBinop(blind,
Token.AND,
createVar(blind, "b", createUnionType(STRING_TYPE, NULL_TYPE)),
createVar(blind, "a", createUnionType(NUMBER_TYPE, VOID_TYPE)),
Sets.newHashSet(new TypedName("a", NUMBER_TYPE),
new TypedName("b", STRING_TYPE)),
Sets.newHashSet(new TypedName("a",
createUnionType(NUMBER_TYPE, VOID_TYPE)),
new TypedName("b",
createUnionType(STRING_TYPE, NULL_TYPE))));
}
@SuppressWarnings("unchecked")
public void testTypeof1() {
FlowScope blind = newScope();
testBinop(blind,
Token.EQ,
new Node(Token.TYPEOF, createVar(blind, "a", OBJECT_TYPE)),
Node.newString("function"),
Sets.newHashSet(
new TypedName("a", U2U_CONSTRUCTOR_TYPE)),
Sets.newHashSet(
new TypedName("a", OBJECT_TYPE)));
}
@SuppressWarnings("unchecked")
public void testTypeof2() {
FlowScope blind = newScope();
testBinop(blind,
Token.EQ,
new Node(Token.TYPEOF, createVar(blind, "a", ALL_TYPE)),
Node.newString("function"),
Sets.newHashSet(
new TypedName("a", U2U_CONSTRUCTOR_TYPE)),
Sets.newHashSet(
new TypedName("a", ALL_TYPE)));
}
@SuppressWarnings("unchecked")
public void testInstanceOf() {
FlowScope blind = newScope();
testBinop(blind,
Token.INSTANCEOF,
createVar(blind, "x", UNKNOWN_TYPE),
createVar(blind, "s", STRING_OBJECT_FUNCTION_TYPE),
Sets.newHashSet(
new TypedName("x", STRING_OBJECT_TYPE),
new TypedName("s", STRING_OBJECT_FUNCTION_TYPE)),
Sets.newHashSet(
new TypedName("s", STRING_OBJECT_FUNCTION_TYPE)));
}
@SuppressWarnings("unchecked")
public void testInstanceOf2() {
FlowScope blind = newScope();
testBinop(blind,
Token.INSTANCEOF,
createVar(blind, "x",
createUnionType(STRING_OBJECT_TYPE, NUMBER_OBJECT_TYPE)),
createVar(blind, "s", STRING_OBJECT_FUNCTION_TYPE),
Sets.newHashSet(
new TypedName("x", STRING_OBJECT_TYPE),
new TypedName("s", STRING_OBJECT_FUNCTION_TYPE)),
Sets.newHashSet(
new TypedName("x", NUMBER_OBJECT_TYPE),
new TypedName("s", STRING_OBJECT_FUNCTION_TYPE)));
}
@SuppressWarnings("unchecked")
public void testInstanceOf3() {
FlowScope blind = newScope();
testBinop(blind,
Token.INSTANCEOF,
createVar(blind, "x", OBJECT_TYPE),
createVar(blind, "s", STRING_OBJECT_FUNCTION_TYPE),
Sets.newHashSet(
new TypedName("x", STRING_OBJECT_TYPE),
new TypedName("s", STRING_OBJECT_FUNCTION_TYPE)),
Sets.newHashSet(
new TypedName("x", OBJECT_TYPE),
new TypedName("s", STRING_OBJECT_FUNCTION_TYPE)));
}
@SuppressWarnings("unchecked")
public void testInstanceOf4() {
FlowScope blind = newScope();
testBinop(blind,
Token.INSTANCEOF,
createVar(blind, "x", ALL_TYPE),
createVar(blind, "s", STRING_OBJECT_FUNCTION_TYPE),
Sets.newHashSet(
new TypedName("x", STRING_OBJECT_TYPE),
new TypedName("s", STRING_OBJECT_FUNCTION_TYPE)),
Sets.newHashSet(
new TypedName("s", STRING_OBJECT_FUNCTION_TYPE)));
}
private void testBinop(FlowScope blind, int binop, Node left, Node right,
Collection<TypedName> trueOutcome,
Collection<TypedName> falseOutcome) {
Node condition = new Node(binop);
condition.addChildToBack(left);
condition.addChildToBack(right);
// true outcome.
FlowScope informedTrue = interpreter.
getPreciserScopeKnowingConditionOutcome(condition, blind, true);
for (TypedName p : trueOutcome) {
assertEquals(p.name, p.type, getVarType(informedTrue, p.name));
}
// false outcome.
FlowScope informedFalse = interpreter.
getPreciserScopeKnowingConditionOutcome(condition, blind, false);
for (TypedName p : falseOutcome) {
assertEquals(p.type, getVarType(informedFalse, p.name));
}
}
private Node createNull() {
Node n = new Node(Token.NULL);
n.setJSType(NULL_TYPE);
return n;
}
private Node createNumber(int n) {
Node number = createUntypedNumber(n);
number.setJSType(NUMBER_TYPE);
return number;
}
private Node createUntypedNumber(int n) {
return Node.newNumber(n);
}
private JSType getVarType(FlowScope scope, String name) {
return scope.getSlot(name).getType();
}
private Node createVar(FlowScope scope, String name, JSType type) {
Node n = Node.newString(Token.NAME, name);
functionScope.declare(name, n, null, null);
((LinkedFlowScope) scope).inferSlotType(name, type);
n.setJSType(type);
return n;
}
private static class TypedName {
private final String name;
private final JSType type;
private TypedName(String name, JSType type) {
this.name = name;
this.type = type;
}
}
} |
|
public void testIssue115() {
CompilerRunner.FLAG_compilation_level.setForTest(
CompilationLevel.SIMPLE_OPTIMIZATIONS);
CompilerRunner.FLAG_warning_level.setForTest(
WarningLevel.VERBOSE);
test("function f() { " +
" var arguments = Array.prototype.slice.call(arguments, 0);" +
" return arguments[0]; " +
"}",
"function f() { " +
" arguments = Array.prototype.slice.call(arguments, 0);" +
" return arguments[0]; " +
"}");
} | com.google.javascript.jscomp.CompilerRunnerTest::testIssue115 | test/com/google/javascript/jscomp/CompilerRunnerTest.java | 193 | test/com/google/javascript/jscomp/CompilerRunnerTest.java | testIssue115 | /*
* Copyright 2009 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import com.google.common.base.Joiner;
import com.google.common.collect.Lists;
import com.google.common.flags.Flags;
import com.google.javascript.rhino.Node;
import junit.framework.TestCase;
import java.io.IOException;
/**
* Tests for {@link CompilerRunner}.
*
*
*/
public class CompilerRunnerTest extends TestCase {
private Compiler lastCompiler = null;
// If set to true, uses comparison by string instead of by AST.
private boolean useStringComparison = false;
/** Externs for the test */
private final JSSourceFile[] externs = new JSSourceFile[] {
JSSourceFile.fromCode("externs",
"var arguments;" +
"/** @constructor \n * @param {...*} var_args \n " +
"* @return {!Array} */ " +
"function Array(var_args) {}\n"
+ "/** @constructor */ function Window() {}\n"
+ "/** @type {string} */ Window.prototype.name;\n"
+ "/** @type {Window} */ var window;"
+ "/** @nosideeffects */ function noSideEffects() {}")
};
@Override
public void setUp() throws Exception {
super.setUp();
Flags.disableStateCheckingForTest();
Flags.resetAllFlagsForTest();
lastCompiler = null;
useStringComparison = false;
}
@Override
public void tearDown() throws Exception {
Flags.resetAllFlagsForTest();
// NOTE(nicksantos): ANT needs this for some weird reason.
AbstractCompilerRunner.FLAG_define.resetForTest();
AbstractCompilerRunner.FLAG_jscomp_off.resetForTest();
AbstractCompilerRunner.FLAG_jscomp_warning.resetForTest();
AbstractCompilerRunner.FLAG_jscomp_error.resetForTest();
Flags.enableStateCheckingForTest();
super.tearDown();
}
public void testTypeCheckingOffByDefault() {}
// Defects4J: flaky method
// public void testTypeCheckingOffByDefault() {
// test("function f(x) { return x; } f();",
// "function f(a) { return a; } f();");
// }
public void testTypeCheckingOnWithVerbose() {
CompilerRunner.FLAG_warning_level.setForTest(WarningLevel.VERBOSE);
test("function f(x) { return x; } f();", TypeCheck.WRONG_ARGUMENT_COUNT);
}
public void testTypeCheckOverride1() {
CompilerRunner.FLAG_warning_level.setForTest(WarningLevel.VERBOSE);
CompilerRunner.FLAG_jscomp_off.setForTest(
Lists.newArrayList("checkTypes"));
testSame("var x = x || {}; x.f = function() {}; x.f(3);");
}
public void testTypeCheckOverride2() {
CompilerRunner.FLAG_warning_level.setForTest(WarningLevel.DEFAULT);
testSame("var x = x || {}; x.f = function() {}; x.f(3);");
CompilerRunner.FLAG_jscomp_warning.setForTest(
Lists.newArrayList("checkTypes"));
test("var x = x || {}; x.f = function() {}; x.f(3);",
TypeCheck.WRONG_ARGUMENT_COUNT);
}
public void testCheckSymbolsOffForDefault() {
CompilerRunner.FLAG_warning_level.setForTest(WarningLevel.DEFAULT);
test("x = 3; var y; var y;", "x=3; var y;");
}
public void testCheckSymbolsOnForVerbose() {
CompilerRunner.FLAG_warning_level.setForTest(WarningLevel.VERBOSE);
test("x = 3;", VarCheck.UNDEFINED_VAR_ERROR);
test("var y; var y;", SyntacticScopeCreator.VAR_MULTIPLY_DECLARED_ERROR);
}
public void testCheckSymbolsOverrideForVerbose() {
CompilerRunner.FLAG_warning_level.setForTest(WarningLevel.VERBOSE);
AbstractCompilerRunner.FLAG_jscomp_off.setForTest(
Lists.newArrayList("undefinedVars"));
testSame("x = 3;");
}
public void testCheckUndefinedProperties() {
CompilerRunner.FLAG_warning_level.setForTest(WarningLevel.VERBOSE);
AbstractCompilerRunner.FLAG_jscomp_error.setForTest(
Lists.newArrayList("missingProperties"));
test("var x = {}; var y = x.bar;", TypeCheck.INEXISTENT_PROPERTY);
}
public void testDuplicateParams() {
test("function (a, a) {}", RhinoErrorReporter.DUPLICATE_PARAM);
assertTrue(lastCompiler.hasHaltingErrors());
}
public void testDefineFlag() {
AbstractCompilerRunner.FLAG_define.setForTest(
Lists.newArrayList("FOO", "BAR=5"));
test("/** @define {boolean} */ var FOO = false;" +
"/** @define {number} */ var BAR = 3;",
"var FOO = true, BAR = 5;");
}
public void testScriptStrictModeNoWarning() {
test("'use strict';", "");
test("'no use strict';", CheckSideEffects.USELESS_CODE_ERROR);
}
public void testFunctionStrictModeNoWarning() {
test("function f() {'use strict';}", "function f() {}");
test("function f() {'no use strict';}",
CheckSideEffects.USELESS_CODE_ERROR);
}
public void testQuietMode() {}
// Defects4J: flaky method
// public void testQuietMode() {
// CompilerRunner.FLAG_warning_level.setForTest(WarningLevel.DEFAULT);
// test("/** @type { not a type name } */ var x;",
// RhinoErrorReporter.PARSE_ERROR);
// CompilerRunner.FLAG_warning_level.setForTest(WarningLevel.QUIET);
// testSame("/** @type { not a type name } */ var x;");
// }
//////////////////////////////////////////////////////////////////////////////
// Integration tests
public void testIssue70() {
test("function foo({}) {}", RhinoErrorReporter.PARSE_ERROR);
}
public void testIssue81() {}
// Defects4J: flaky method
// public void testIssue81() {
// CompilerRunner.FLAG_compilation_level.setForTest(
// CompilationLevel.ADVANCED_OPTIMIZATIONS);
// useStringComparison = true;
// test("eval('1'); var x = eval; x('2');",
// "eval(\"1\");(0,eval)(\"2\");");
// }
public void testIssue115() {
CompilerRunner.FLAG_compilation_level.setForTest(
CompilationLevel.SIMPLE_OPTIMIZATIONS);
CompilerRunner.FLAG_warning_level.setForTest(
WarningLevel.VERBOSE);
test("function f() { " +
" var arguments = Array.prototype.slice.call(arguments, 0);" +
" return arguments[0]; " +
"}",
"function f() { " +
" arguments = Array.prototype.slice.call(arguments, 0);" +
" return arguments[0]; " +
"}");
}
public void testDebugFlag1() {
CompilerRunner.FLAG_compilation_level.setForTest(
CompilationLevel.SIMPLE_OPTIMIZATIONS);
CompilerRunner.FLAG_debug.setForTest(false);
testSame("function foo(a) {}");
}
public void testDebugFlag2() {
CompilerRunner.FLAG_compilation_level.setForTest(
CompilationLevel.SIMPLE_OPTIMIZATIONS);
CompilerRunner.FLAG_debug.setForTest(true);
test("function foo(a) {}",
"function foo($a$$) {}");
}
public void testDebugFlag3() {
CompilerRunner.FLAG_compilation_level.setForTest(
CompilationLevel.ADVANCED_OPTIMIZATIONS);
CompilerRunner.FLAG_warning_level.setForTest(
WarningLevel.QUIET);
CompilerRunner.FLAG_debug.setForTest(false);
test("function Foo() {};" +
"Foo.x = 1;" +
"function f() {throw new Foo().x;} f();",
"function a() {};" +
"throw new a().a;");
}
public void testDebugFlag4() {
CompilerRunner.FLAG_compilation_level.setForTest(
CompilationLevel.ADVANCED_OPTIMIZATIONS);
CompilerRunner.FLAG_warning_level.setForTest(
WarningLevel.QUIET);
CompilerRunner.FLAG_debug.setForTest(true);
test("function Foo() {};" +
"Foo.x = 1;" +
"function f() {throw new Foo().x;} f();",
"function $Foo$$() {};" +
"throw new $Foo$$().$x$;");
}
/* Helper functions */
private void testSame(String original) {
testSame(new String[] { original });
}
private void testSame(String[] original) {
test(original, original);
}
private void test(String original, String compiled) {
test(new String[] { original }, new String[] { compiled });
}
/**
* Asserts that when compiling with the given compiler options,
* {@code original} is transformed into {@code compiled}.
*/
private void test(String[] original, String[] compiled) {
Compiler compiler = compile(original);
assertEquals("Expected no warnings or errors\n" +
"Errors: \n" + Joiner.on("\n").join(compiler.getErrors()) +
"Warnings: \n" + Joiner.on("\n").join(compiler.getWarnings()),
0, compiler.getErrors().length + compiler.getWarnings().length);
Node root = compiler.getRoot().getLastChild();
if (useStringComparison) {
assertEquals(Joiner.on("").join(compiled), compiler.toSource());
} else {
Node expectedRoot = parse(compiled);
String explanation = expectedRoot.checkTreeEquals(root);
assertNull("\nExpected: " + compiler.toSource(expectedRoot) +
"\nResult: " + compiler.toSource(root) +
"\n" + explanation, explanation);
}
}
/**
* Asserts that when compiling, there is an error or warning.
*/
private void test(String original, DiagnosticType warning) {
test(new String[] { original }, warning);
}
/**
* Asserts that when compiling, there is an error or warning.
*/
private void test(String[] original, DiagnosticType warning) {
Compiler compiler = compile(original);
assertEquals("Expected exactly one warning or error " +
"Errors: \n" + Joiner.on("\n").join(compiler.getErrors()) +
"Warnings: \n" + Joiner.on("\n").join(compiler.getWarnings()),
1, compiler.getErrors().length + compiler.getWarnings().length);
if (compiler.getErrors().length > 0) {
assertEquals(warning, compiler.getErrors()[0].getType());
} else {
assertEquals(warning, compiler.getWarnings()[0].getType());
}
}
private Compiler compile(String original) {
return compile( new String[] { original });
}
private Compiler compile(String[] original) {
CompilerRunner runner = new CompilerRunner(new String[] {});
Compiler compiler = runner.createCompiler();
lastCompiler = compiler;
JSSourceFile[] inputs = new JSSourceFile[original.length];
for (int i = 0; i < original.length; i++) {
inputs[i] = JSSourceFile.fromCode("input" + i, original[i]);
}
CompilerOptions options = runner.createOptions();
try {
runner.setRunOptions(options);
} catch (AbstractCompilerRunner.FlagUsageException e) {
fail("Unexpected exception " + e);
} catch (IOException e) {
assert(false);
}
compiler.compile(
externs, CompilerTestCase.createModuleChain(original), options);
return compiler;
}
private Node parse(String[] original) {
CompilerRunner runner = new CompilerRunner(new String[] {});
Compiler compiler = runner.createCompiler();
JSSourceFile[] inputs = new JSSourceFile[original.length];
for (int i = 0; i < inputs.length; i++) {
inputs[i] = JSSourceFile.fromCode("input" + i, original[i]);
}
compiler.init(externs, inputs, new CompilerOptions());
Node all = compiler.parseInputs();
Node n = all.getLastChild();
return n;
}
} | // You are a professional Java test case writer, please create a test case named `testIssue115` for the issue `Closure-115`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-115
//
// ## Issue-Title:
// compiler assumes that 'arguments' can be shadowed
//
// ## Issue-Description:
// The code:
// function name() {
// var arguments = Array.prototype.slice.call(arguments, 0);
// }
//
// gets compiled to:
// function name(){ var c=Array.prototype.slice.call(c,0); }
//
// Thanks to tescosquirrel for the report.
//
//
public void testIssue115() {
| 193 | // }
// "eval(\"1\");(0,eval)(\"2\");");
// test("eval('1'); var x = eval; x('2');",
// useStringComparison = true;
// CompilationLevel.ADVANCED_OPTIMIZATIONS);
// CompilerRunner.FLAG_compilation_level.setForTest(
// public void testIssue81() {
// Defects4J: flaky method | 102 | 180 | test/com/google/javascript/jscomp/CompilerRunnerTest.java | test | ```markdown
## Issue-ID: Closure-115
## Issue-Title:
compiler assumes that 'arguments' can be shadowed
## Issue-Description:
The code:
function name() {
var arguments = Array.prototype.slice.call(arguments, 0);
}
gets compiled to:
function name(){ var c=Array.prototype.slice.call(c,0); }
Thanks to tescosquirrel for the report.
```
You are a professional Java test case writer, please create a test case named `testIssue115` for the issue `Closure-115`, utilizing the provided issue report information and the following function signature.
```java
public void testIssue115() {
```
| 180 | [
"com.google.javascript.jscomp.Normalize"
] | 87704a4e3942c73cc13aed27b67f8a3f09be4324cecf85d28214f2bf2dbbb958 | public void testIssue115() | // You are a professional Java test case writer, please create a test case named `testIssue115` for the issue `Closure-115`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-115
//
// ## Issue-Title:
// compiler assumes that 'arguments' can be shadowed
//
// ## Issue-Description:
// The code:
// function name() {
// var arguments = Array.prototype.slice.call(arguments, 0);
// }
//
// gets compiled to:
// function name(){ var c=Array.prototype.slice.call(c,0); }
//
// Thanks to tescosquirrel for the report.
//
//
| Closure | /*
* Copyright 2009 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import com.google.common.base.Joiner;
import com.google.common.collect.Lists;
import com.google.common.flags.Flags;
import com.google.javascript.rhino.Node;
import junit.framework.TestCase;
import java.io.IOException;
/**
* Tests for {@link CompilerRunner}.
*
*
*/
public class CompilerRunnerTest extends TestCase {
private Compiler lastCompiler = null;
// If set to true, uses comparison by string instead of by AST.
private boolean useStringComparison = false;
/** Externs for the test */
private final JSSourceFile[] externs = new JSSourceFile[] {
JSSourceFile.fromCode("externs",
"var arguments;" +
"/** @constructor \n * @param {...*} var_args \n " +
"* @return {!Array} */ " +
"function Array(var_args) {}\n"
+ "/** @constructor */ function Window() {}\n"
+ "/** @type {string} */ Window.prototype.name;\n"
+ "/** @type {Window} */ var window;"
+ "/** @nosideeffects */ function noSideEffects() {}")
};
@Override
public void setUp() throws Exception {
super.setUp();
Flags.disableStateCheckingForTest();
Flags.resetAllFlagsForTest();
lastCompiler = null;
useStringComparison = false;
}
@Override
public void tearDown() throws Exception {
Flags.resetAllFlagsForTest();
// NOTE(nicksantos): ANT needs this for some weird reason.
AbstractCompilerRunner.FLAG_define.resetForTest();
AbstractCompilerRunner.FLAG_jscomp_off.resetForTest();
AbstractCompilerRunner.FLAG_jscomp_warning.resetForTest();
AbstractCompilerRunner.FLAG_jscomp_error.resetForTest();
Flags.enableStateCheckingForTest();
super.tearDown();
}
public void testTypeCheckingOffByDefault() {}
// Defects4J: flaky method
// public void testTypeCheckingOffByDefault() {
// test("function f(x) { return x; } f();",
// "function f(a) { return a; } f();");
// }
public void testTypeCheckingOnWithVerbose() {
CompilerRunner.FLAG_warning_level.setForTest(WarningLevel.VERBOSE);
test("function f(x) { return x; } f();", TypeCheck.WRONG_ARGUMENT_COUNT);
}
public void testTypeCheckOverride1() {
CompilerRunner.FLAG_warning_level.setForTest(WarningLevel.VERBOSE);
CompilerRunner.FLAG_jscomp_off.setForTest(
Lists.newArrayList("checkTypes"));
testSame("var x = x || {}; x.f = function() {}; x.f(3);");
}
public void testTypeCheckOverride2() {
CompilerRunner.FLAG_warning_level.setForTest(WarningLevel.DEFAULT);
testSame("var x = x || {}; x.f = function() {}; x.f(3);");
CompilerRunner.FLAG_jscomp_warning.setForTest(
Lists.newArrayList("checkTypes"));
test("var x = x || {}; x.f = function() {}; x.f(3);",
TypeCheck.WRONG_ARGUMENT_COUNT);
}
public void testCheckSymbolsOffForDefault() {
CompilerRunner.FLAG_warning_level.setForTest(WarningLevel.DEFAULT);
test("x = 3; var y; var y;", "x=3; var y;");
}
public void testCheckSymbolsOnForVerbose() {
CompilerRunner.FLAG_warning_level.setForTest(WarningLevel.VERBOSE);
test("x = 3;", VarCheck.UNDEFINED_VAR_ERROR);
test("var y; var y;", SyntacticScopeCreator.VAR_MULTIPLY_DECLARED_ERROR);
}
public void testCheckSymbolsOverrideForVerbose() {
CompilerRunner.FLAG_warning_level.setForTest(WarningLevel.VERBOSE);
AbstractCompilerRunner.FLAG_jscomp_off.setForTest(
Lists.newArrayList("undefinedVars"));
testSame("x = 3;");
}
public void testCheckUndefinedProperties() {
CompilerRunner.FLAG_warning_level.setForTest(WarningLevel.VERBOSE);
AbstractCompilerRunner.FLAG_jscomp_error.setForTest(
Lists.newArrayList("missingProperties"));
test("var x = {}; var y = x.bar;", TypeCheck.INEXISTENT_PROPERTY);
}
public void testDuplicateParams() {
test("function (a, a) {}", RhinoErrorReporter.DUPLICATE_PARAM);
assertTrue(lastCompiler.hasHaltingErrors());
}
public void testDefineFlag() {
AbstractCompilerRunner.FLAG_define.setForTest(
Lists.newArrayList("FOO", "BAR=5"));
test("/** @define {boolean} */ var FOO = false;" +
"/** @define {number} */ var BAR = 3;",
"var FOO = true, BAR = 5;");
}
public void testScriptStrictModeNoWarning() {
test("'use strict';", "");
test("'no use strict';", CheckSideEffects.USELESS_CODE_ERROR);
}
public void testFunctionStrictModeNoWarning() {
test("function f() {'use strict';}", "function f() {}");
test("function f() {'no use strict';}",
CheckSideEffects.USELESS_CODE_ERROR);
}
public void testQuietMode() {}
// Defects4J: flaky method
// public void testQuietMode() {
// CompilerRunner.FLAG_warning_level.setForTest(WarningLevel.DEFAULT);
// test("/** @type { not a type name } */ var x;",
// RhinoErrorReporter.PARSE_ERROR);
// CompilerRunner.FLAG_warning_level.setForTest(WarningLevel.QUIET);
// testSame("/** @type { not a type name } */ var x;");
// }
//////////////////////////////////////////////////////////////////////////////
// Integration tests
public void testIssue70() {
test("function foo({}) {}", RhinoErrorReporter.PARSE_ERROR);
}
public void testIssue81() {}
// Defects4J: flaky method
// public void testIssue81() {
// CompilerRunner.FLAG_compilation_level.setForTest(
// CompilationLevel.ADVANCED_OPTIMIZATIONS);
// useStringComparison = true;
// test("eval('1'); var x = eval; x('2');",
// "eval(\"1\");(0,eval)(\"2\");");
// }
public void testIssue115() {
CompilerRunner.FLAG_compilation_level.setForTest(
CompilationLevel.SIMPLE_OPTIMIZATIONS);
CompilerRunner.FLAG_warning_level.setForTest(
WarningLevel.VERBOSE);
test("function f() { " +
" var arguments = Array.prototype.slice.call(arguments, 0);" +
" return arguments[0]; " +
"}",
"function f() { " +
" arguments = Array.prototype.slice.call(arguments, 0);" +
" return arguments[0]; " +
"}");
}
public void testDebugFlag1() {
CompilerRunner.FLAG_compilation_level.setForTest(
CompilationLevel.SIMPLE_OPTIMIZATIONS);
CompilerRunner.FLAG_debug.setForTest(false);
testSame("function foo(a) {}");
}
public void testDebugFlag2() {
CompilerRunner.FLAG_compilation_level.setForTest(
CompilationLevel.SIMPLE_OPTIMIZATIONS);
CompilerRunner.FLAG_debug.setForTest(true);
test("function foo(a) {}",
"function foo($a$$) {}");
}
public void testDebugFlag3() {
CompilerRunner.FLAG_compilation_level.setForTest(
CompilationLevel.ADVANCED_OPTIMIZATIONS);
CompilerRunner.FLAG_warning_level.setForTest(
WarningLevel.QUIET);
CompilerRunner.FLAG_debug.setForTest(false);
test("function Foo() {};" +
"Foo.x = 1;" +
"function f() {throw new Foo().x;} f();",
"function a() {};" +
"throw new a().a;");
}
public void testDebugFlag4() {
CompilerRunner.FLAG_compilation_level.setForTest(
CompilationLevel.ADVANCED_OPTIMIZATIONS);
CompilerRunner.FLAG_warning_level.setForTest(
WarningLevel.QUIET);
CompilerRunner.FLAG_debug.setForTest(true);
test("function Foo() {};" +
"Foo.x = 1;" +
"function f() {throw new Foo().x;} f();",
"function $Foo$$() {};" +
"throw new $Foo$$().$x$;");
}
/* Helper functions */
private void testSame(String original) {
testSame(new String[] { original });
}
private void testSame(String[] original) {
test(original, original);
}
private void test(String original, String compiled) {
test(new String[] { original }, new String[] { compiled });
}
/**
* Asserts that when compiling with the given compiler options,
* {@code original} is transformed into {@code compiled}.
*/
private void test(String[] original, String[] compiled) {
Compiler compiler = compile(original);
assertEquals("Expected no warnings or errors\n" +
"Errors: \n" + Joiner.on("\n").join(compiler.getErrors()) +
"Warnings: \n" + Joiner.on("\n").join(compiler.getWarnings()),
0, compiler.getErrors().length + compiler.getWarnings().length);
Node root = compiler.getRoot().getLastChild();
if (useStringComparison) {
assertEquals(Joiner.on("").join(compiled), compiler.toSource());
} else {
Node expectedRoot = parse(compiled);
String explanation = expectedRoot.checkTreeEquals(root);
assertNull("\nExpected: " + compiler.toSource(expectedRoot) +
"\nResult: " + compiler.toSource(root) +
"\n" + explanation, explanation);
}
}
/**
* Asserts that when compiling, there is an error or warning.
*/
private void test(String original, DiagnosticType warning) {
test(new String[] { original }, warning);
}
/**
* Asserts that when compiling, there is an error or warning.
*/
private void test(String[] original, DiagnosticType warning) {
Compiler compiler = compile(original);
assertEquals("Expected exactly one warning or error " +
"Errors: \n" + Joiner.on("\n").join(compiler.getErrors()) +
"Warnings: \n" + Joiner.on("\n").join(compiler.getWarnings()),
1, compiler.getErrors().length + compiler.getWarnings().length);
if (compiler.getErrors().length > 0) {
assertEquals(warning, compiler.getErrors()[0].getType());
} else {
assertEquals(warning, compiler.getWarnings()[0].getType());
}
}
private Compiler compile(String original) {
return compile( new String[] { original });
}
private Compiler compile(String[] original) {
CompilerRunner runner = new CompilerRunner(new String[] {});
Compiler compiler = runner.createCompiler();
lastCompiler = compiler;
JSSourceFile[] inputs = new JSSourceFile[original.length];
for (int i = 0; i < original.length; i++) {
inputs[i] = JSSourceFile.fromCode("input" + i, original[i]);
}
CompilerOptions options = runner.createOptions();
try {
runner.setRunOptions(options);
} catch (AbstractCompilerRunner.FlagUsageException e) {
fail("Unexpected exception " + e);
} catch (IOException e) {
assert(false);
}
compiler.compile(
externs, CompilerTestCase.createModuleChain(original), options);
return compiler;
}
private Node parse(String[] original) {
CompilerRunner runner = new CompilerRunner(new String[] {});
Compiler compiler = runner.createCompiler();
JSSourceFile[] inputs = new JSSourceFile[original.length];
for (int i = 0; i < inputs.length; i++) {
inputs[i] = JSSourceFile.fromCode("input" + i, original[i]);
}
compiler.init(externs, inputs, new CompilerOptions());
Node all = compiler.parseInputs();
Node n = all.getLastChild();
return n;
}
} |
|
public void testToClass_object() {
assertNull(ClassUtils.toClass(null));
assertSame(ArrayUtils.EMPTY_CLASS_ARRAY, ClassUtils.toClass(ArrayUtils.EMPTY_OBJECT_ARRAY));
assertTrue(Arrays.equals(new Class[] { String.class, Integer.class, Double.class },
ClassUtils.toClass(new Object[] { "Test", 1, 99d })));
assertTrue(Arrays.equals(new Class[] { String.class, null, Double.class },
ClassUtils.toClass(new Object[] { "Test", null, 99d })));
} | org.apache.commons.lang3.ClassUtilsTest::testToClass_object | src/test/java/org/apache/commons/lang3/ClassUtilsTest.java | 911 | src/test/java/org/apache/commons/lang3/ClassUtilsTest.java | testToClass_object | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.lang3;
import java.lang.reflect.Constructor;
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import junit.framework.TestCase;
/**
* Unit tests {@link org.apache.commons.lang3.ClassUtils}.
*
* @author Apache Software Foundation
* @author Gary D. Gregory
* @author Tomasz Blachowicz
* @version $Id$
*/
public class ClassUtilsTest extends TestCase {
public ClassUtilsTest(String name) {
super(name);
}
private static class Inner {
}
//-----------------------------------------------------------------------
public void testConstructor() {
assertNotNull(new ClassUtils());
Constructor<?>[] cons = ClassUtils.class.getDeclaredConstructors();
assertEquals(1, cons.length);
assertEquals(true, Modifier.isPublic(cons[0].getModifiers()));
assertEquals(true, Modifier.isPublic(ClassUtils.class.getModifiers()));
assertEquals(false, Modifier.isFinal(ClassUtils.class.getModifiers()));
}
// -------------------------------------------------------------------------
public void test_getShortClassName_Object() {
assertEquals("ClassUtils", ClassUtils.getShortClassName(new ClassUtils(), "<null>"));
assertEquals("ClassUtilsTest.Inner", ClassUtils.getShortClassName(new Inner(), "<null>"));
assertEquals("String", ClassUtils.getShortClassName("hello", "<null>"));
assertEquals("<null>", ClassUtils.getShortClassName(null, "<null>"));
}
public void test_getShortClassName_Class() {
assertEquals("ClassUtils", ClassUtils.getShortClassName(ClassUtils.class));
assertEquals("Map.Entry", ClassUtils.getShortClassName(Map.Entry.class));
assertEquals("", ClassUtils.getShortClassName((Class<?>) null));
// LANG-535
assertEquals("String[]", ClassUtils.getShortClassName(String[].class));
assertEquals("Map.Entry[]", ClassUtils.getShortClassName(Map.Entry[].class));
// Primitives
assertEquals("boolean", ClassUtils.getShortClassName(boolean.class));
assertEquals("byte", ClassUtils.getShortClassName(byte.class));
assertEquals("char", ClassUtils.getShortClassName(char.class));
assertEquals("short", ClassUtils.getShortClassName(short.class));
assertEquals("int", ClassUtils.getShortClassName(int.class));
assertEquals("long", ClassUtils.getShortClassName(long.class));
assertEquals("float", ClassUtils.getShortClassName(float.class));
assertEquals("double", ClassUtils.getShortClassName(double.class));
// Primitive Arrays
assertEquals("boolean[]", ClassUtils.getShortClassName(boolean[].class));
assertEquals("byte[]", ClassUtils.getShortClassName(byte[].class));
assertEquals("char[]", ClassUtils.getShortClassName(char[].class));
assertEquals("short[]", ClassUtils.getShortClassName(short[].class));
assertEquals("int[]", ClassUtils.getShortClassName(int[].class));
assertEquals("long[]", ClassUtils.getShortClassName(long[].class));
assertEquals("float[]", ClassUtils.getShortClassName(float[].class));
assertEquals("double[]", ClassUtils.getShortClassName(double[].class));
// Arrays of arrays of ...
assertEquals("String[][]", ClassUtils.getShortClassName(String[][].class));
assertEquals("String[][][]", ClassUtils.getShortClassName(String[][][].class));
assertEquals("String[][][][]", ClassUtils.getShortClassName(String[][][][].class));
}
public void test_getShortClassName_String() {
assertEquals("ClassUtils", ClassUtils.getShortClassName(ClassUtils.class.getName()));
assertEquals("Map.Entry", ClassUtils.getShortClassName(Map.Entry.class.getName()));
assertEquals("", ClassUtils.getShortClassName((String) null));
assertEquals("", ClassUtils.getShortClassName(""));
}
// -------------------------------------------------------------------------
public void test_getPackageName_Object() {
assertEquals("org.apache.commons.lang3", ClassUtils.getPackageName(new ClassUtils(), "<null>"));
assertEquals("org.apache.commons.lang3", ClassUtils.getPackageName(new Inner(), "<null>"));
assertEquals("<null>", ClassUtils.getPackageName(null, "<null>"));
}
public void test_getPackageName_Class() {
assertEquals("java.lang", ClassUtils.getPackageName(String.class));
assertEquals("java.util", ClassUtils.getPackageName(Map.Entry.class));
assertEquals("", ClassUtils.getPackageName((Class<?>)null));
// LANG-535
assertEquals("java.lang", ClassUtils.getPackageName(String[].class));
// Primitive Arrays
assertEquals("", ClassUtils.getPackageName(boolean[].class));
assertEquals("", ClassUtils.getPackageName(byte[].class));
assertEquals("", ClassUtils.getPackageName(char[].class));
assertEquals("", ClassUtils.getPackageName(short[].class));
assertEquals("", ClassUtils.getPackageName(int[].class));
assertEquals("", ClassUtils.getPackageName(long[].class));
assertEquals("", ClassUtils.getPackageName(float[].class));
assertEquals("", ClassUtils.getPackageName(double[].class));
// Arrays of arrays of ...
assertEquals("java.lang", ClassUtils.getPackageName(String[][].class));
assertEquals("java.lang", ClassUtils.getPackageName(String[][][].class));
assertEquals("java.lang", ClassUtils.getPackageName(String[][][][].class));
}
public void test_getPackageName_String() {
assertEquals("org.apache.commons.lang3", ClassUtils.getPackageName(ClassUtils.class.getName()));
assertEquals("java.util", ClassUtils.getPackageName(Map.Entry.class.getName()));
assertEquals("", ClassUtils.getPackageName((String)null));
assertEquals("", ClassUtils.getPackageName(""));
}
// -------------------------------------------------------------------------
public void test_getAllSuperclasses_Class() {
List<?> list = ClassUtils.getAllSuperclasses(CY.class);
assertEquals(2, list.size());
assertEquals(CX.class, list.get(0));
assertEquals(Object.class, list.get(1));
assertEquals(null, ClassUtils.getAllSuperclasses(null));
}
public void test_getAllInterfaces_Class() {
List<?> list = ClassUtils.getAllInterfaces(CY.class);
assertEquals(6, list.size());
assertEquals(IB.class, list.get(0));
assertEquals(IC.class, list.get(1));
assertEquals(ID.class, list.get(2));
assertEquals(IE.class, list.get(3));
assertEquals(IF.class, list.get(4));
assertEquals(IA.class, list.get(5));
assertEquals(null, ClassUtils.getAllInterfaces(null));
}
private static interface IA {
}
private static interface IB {
}
private static interface IC extends ID, IE {
}
private static interface ID {
}
private static interface IE extends IF {
}
private static interface IF {
}
private static class CX implements IB, IA, IE {
}
private static class CY extends CX implements IB, IC {
}
// -------------------------------------------------------------------------
public void test_convertClassNamesToClasses_List() {
List<String> list = new ArrayList<String>();
List<Class<?>> result = ClassUtils.convertClassNamesToClasses(list);
assertEquals(0, result.size());
list.add("java.lang.String");
list.add("java.lang.xxx");
list.add("java.lang.Object");
result = ClassUtils.convertClassNamesToClasses(list);
assertEquals(3, result.size());
assertEquals(String.class, result.get(0));
assertEquals(null, result.get(1));
assertEquals(Object.class, result.get(2));
@SuppressWarnings("unchecked") // test what happens when non-gneric code adds wrong type of element
List<Object> olist = (List<Object>)(List<?>)list;
olist.add(new Object());
try {
ClassUtils.convertClassNamesToClasses(list);
fail("Should not have been able to convert list");
} catch (ClassCastException expected) {}
assertEquals(null, ClassUtils.convertClassNamesToClasses(null));
}
public void test_convertClassesToClassNames_List() {
List<Class<?>> list = new ArrayList<Class<?>>();
List<String> result = ClassUtils.convertClassesToClassNames(list);
assertEquals(0, result.size());
list.add(String.class);
list.add(null);
list.add(Object.class);
result = ClassUtils.convertClassesToClassNames(list);
assertEquals(3, result.size());
assertEquals("java.lang.String", result.get(0));
assertEquals(null, result.get(1));
assertEquals("java.lang.Object", result.get(2));
@SuppressWarnings("unchecked") // test what happens when non-gneric code adds wrong type of element
List<Object> olist = (List<Object>)(List<?>)list;
olist.add(new Object());
try {
ClassUtils.convertClassesToClassNames(list);
fail("Should not have been able to convert list");
} catch (ClassCastException expected) {}
assertEquals(null, ClassUtils.convertClassesToClassNames(null));
}
// -------------------------------------------------------------------------
public void test_isInnerClass_Class() {
assertEquals(true, ClassUtils.isInnerClass(Inner.class));
assertEquals(true, ClassUtils.isInnerClass(Map.Entry.class));
assertEquals(true, ClassUtils.isInnerClass(new Cloneable() {
}.getClass()));
assertEquals(false, ClassUtils.isInnerClass(this.getClass()));
assertEquals(false, ClassUtils.isInnerClass(String.class));
assertEquals(false, ClassUtils.isInnerClass(null));
}
// -------------------------------------------------------------------------
public void test_isAssignable_ClassArray_ClassArray() throws Exception {
Class<?>[] array2 = new Class[] {Object.class, Object.class};
Class<?>[] array1 = new Class[] {Object.class};
Class<?>[] array1s = new Class[] {String.class};
Class<?>[] array0 = new Class[] {};
Class<?>[] arrayPrimitives = { Integer.TYPE, Boolean.TYPE };
Class<?>[] arrayWrappers = { Integer.class, Boolean.class };
assertFalse(ClassUtils.isAssignable(array1, array2));
assertFalse(ClassUtils.isAssignable(null, array2));
assertTrue(ClassUtils.isAssignable(null, array0));
assertTrue(ClassUtils.isAssignable(array0, array0));
assertTrue(ClassUtils.isAssignable(array0, null));
assertTrue(ClassUtils.isAssignable((Class[]) null, (Class[]) null));
assertFalse(ClassUtils.isAssignable(array1, array1s));
assertTrue(ClassUtils.isAssignable(array1s, array1s));
assertTrue(ClassUtils.isAssignable(array1s, array1));
boolean autoboxing = SystemUtils.isJavaVersionAtLeast(1.5f);
assertEquals(autoboxing, ClassUtils.isAssignable(arrayPrimitives, arrayWrappers));
assertEquals(autoboxing, ClassUtils.isAssignable(arrayWrappers, arrayPrimitives));
assertFalse(ClassUtils.isAssignable(arrayPrimitives, array1));
assertFalse(ClassUtils.isAssignable(arrayWrappers, array1));
assertEquals(autoboxing, ClassUtils.isAssignable(arrayPrimitives, array2));
assertTrue(ClassUtils.isAssignable(arrayWrappers, array2));
}
public void test_isAssignable_ClassArray_ClassArray_Autoboxing() throws Exception {
Class<?>[] array2 = new Class[] {Object.class, Object.class};
Class<?>[] array1 = new Class[] {Object.class};
Class<?>[] array1s = new Class[] {String.class};
Class<?>[] array0 = new Class[] {};
Class<?>[] arrayPrimitives = { Integer.TYPE, Boolean.TYPE };
Class<?>[] arrayWrappers = { Integer.class, Boolean.class };
assertFalse(ClassUtils.isAssignable(array1, array2, true));
assertFalse(ClassUtils.isAssignable(null, array2, true));
assertTrue(ClassUtils.isAssignable(null, array0, true));
assertTrue(ClassUtils.isAssignable(array0, array0, true));
assertTrue(ClassUtils.isAssignable(array0, null, true));
assertTrue(ClassUtils.isAssignable((Class[]) null, (Class[]) null, true));
assertFalse(ClassUtils.isAssignable(array1, array1s, true));
assertTrue(ClassUtils.isAssignable(array1s, array1s, true));
assertTrue(ClassUtils.isAssignable(array1s, array1, true));
assertTrue(ClassUtils.isAssignable(arrayPrimitives, arrayWrappers, true));
assertTrue(ClassUtils.isAssignable(arrayWrappers, arrayPrimitives, true));
assertFalse(ClassUtils.isAssignable(arrayPrimitives, array1, true));
assertFalse(ClassUtils.isAssignable(arrayWrappers, array1, true));
assertTrue(ClassUtils.isAssignable(arrayPrimitives, array2, true));
assertTrue(ClassUtils.isAssignable(arrayWrappers, array2, true));
}
public void test_isAssignable_ClassArray_ClassArray_NoAutoboxing() throws Exception {
Class<?>[] array2 = new Class[] {Object.class, Object.class};
Class<?>[] array1 = new Class[] {Object.class};
Class<?>[] array1s = new Class[] {String.class};
Class<?>[] array0 = new Class[] {};
Class<?>[] arrayPrimitives = { Integer.TYPE, Boolean.TYPE };
Class<?>[] arrayWrappers = { Integer.class, Boolean.class };
assertFalse(ClassUtils.isAssignable(array1, array2, false));
assertFalse(ClassUtils.isAssignable(null, array2, false));
assertTrue(ClassUtils.isAssignable(null, array0, false));
assertTrue(ClassUtils.isAssignable(array0, array0, false));
assertTrue(ClassUtils.isAssignable(array0, null, false));
assertTrue(ClassUtils.isAssignable((Class[]) null, (Class[]) null, false));
assertFalse(ClassUtils.isAssignable(array1, array1s, false));
assertTrue(ClassUtils.isAssignable(array1s, array1s, false));
assertTrue(ClassUtils.isAssignable(array1s, array1, false));
assertFalse(ClassUtils.isAssignable(arrayPrimitives, arrayWrappers, false));
assertFalse(ClassUtils.isAssignable(arrayWrappers, arrayPrimitives, false));
assertFalse(ClassUtils.isAssignable(arrayPrimitives, array1, false));
assertFalse(ClassUtils.isAssignable(arrayWrappers, array1, false));
assertTrue(ClassUtils.isAssignable(arrayWrappers, array2, false));
assertFalse(ClassUtils.isAssignable(arrayPrimitives, array2, false));
}
public void test_isAssignable() throws Exception {
assertFalse(ClassUtils.isAssignable((Class<?>) null, null));
assertFalse(ClassUtils.isAssignable(String.class, null));
assertTrue(ClassUtils.isAssignable(null, Object.class));
assertTrue(ClassUtils.isAssignable(null, Integer.class));
assertFalse(ClassUtils.isAssignable(null, Integer.TYPE));
assertTrue(ClassUtils.isAssignable(String.class, Object.class));
assertTrue(ClassUtils.isAssignable(String.class, String.class));
assertFalse(ClassUtils.isAssignable(Object.class, String.class));
boolean autoboxing = SystemUtils.isJavaVersionAtLeast(1.5f);
assertEquals(autoboxing, ClassUtils.isAssignable(Integer.TYPE, Integer.class));
assertEquals(autoboxing, ClassUtils.isAssignable(Integer.TYPE, Object.class));
assertEquals(autoboxing, ClassUtils.isAssignable(Integer.class, Integer.TYPE));
assertEquals(autoboxing, ClassUtils.isAssignable(Integer.class, Object.class));
assertTrue(ClassUtils.isAssignable(Integer.TYPE, Integer.TYPE));
assertTrue(ClassUtils.isAssignable(Integer.class, Integer.class));
assertEquals(autoboxing, ClassUtils.isAssignable(Boolean.TYPE, Boolean.class));
assertEquals(autoboxing, ClassUtils.isAssignable(Boolean.TYPE, Object.class));
assertEquals(autoboxing, ClassUtils.isAssignable(Boolean.class, Boolean.TYPE));
assertEquals(autoboxing, ClassUtils.isAssignable(Boolean.class, Object.class));
assertTrue(ClassUtils.isAssignable(Boolean.TYPE, Boolean.TYPE));
assertTrue(ClassUtils.isAssignable(Boolean.class, Boolean.class));
}
public void test_isAssignable_Autoboxing() throws Exception {
assertFalse(ClassUtils.isAssignable((Class<?>) null, null, true));
assertFalse(ClassUtils.isAssignable(String.class, null, true));
assertTrue(ClassUtils.isAssignable(null, Object.class, true));
assertTrue(ClassUtils.isAssignable(null, Integer.class, true));
assertFalse(ClassUtils.isAssignable(null, Integer.TYPE, true));
assertTrue(ClassUtils.isAssignable(String.class, Object.class, true));
assertTrue(ClassUtils.isAssignable(String.class, String.class, true));
assertFalse(ClassUtils.isAssignable(Object.class, String.class, true));
assertTrue(ClassUtils.isAssignable(Integer.TYPE, Integer.class, true));
assertTrue(ClassUtils.isAssignable(Integer.TYPE, Object.class, true));
assertTrue(ClassUtils.isAssignable(Integer.class, Integer.TYPE, true));
assertTrue(ClassUtils.isAssignable(Integer.class, Object.class, true));
assertTrue(ClassUtils.isAssignable(Integer.TYPE, Integer.TYPE, true));
assertTrue(ClassUtils.isAssignable(Integer.class, Integer.class, true));
assertTrue(ClassUtils.isAssignable(Boolean.TYPE, Boolean.class, true));
assertTrue(ClassUtils.isAssignable(Boolean.class, Boolean.TYPE, true));
assertTrue(ClassUtils.isAssignable(Boolean.class, Object.class, true));
assertTrue(ClassUtils.isAssignable(Boolean.TYPE, Boolean.TYPE, true));
assertTrue(ClassUtils.isAssignable(Boolean.class, Boolean.class, true));
}
public void test_isAssignable_NoAutoboxing() throws Exception {
assertFalse(ClassUtils.isAssignable((Class<?>) null, null, false));
assertFalse(ClassUtils.isAssignable(String.class, null, false));
assertTrue(ClassUtils.isAssignable(null, Object.class, false));
assertTrue(ClassUtils.isAssignable(null, Integer.class, false));
assertFalse(ClassUtils.isAssignable(null, Integer.TYPE, false));
assertTrue(ClassUtils.isAssignable(String.class, Object.class, false));
assertTrue(ClassUtils.isAssignable(String.class, String.class, false));
assertFalse(ClassUtils.isAssignable(Object.class, String.class, false));
assertFalse(ClassUtils.isAssignable(Integer.TYPE, Integer.class, false));
assertFalse(ClassUtils.isAssignable(Integer.TYPE, Object.class, false));
assertFalse(ClassUtils.isAssignable(Integer.class, Integer.TYPE, false));
assertTrue(ClassUtils.isAssignable(Integer.TYPE, Integer.TYPE, false));
assertTrue(ClassUtils.isAssignable(Integer.class, Integer.class, false));
assertFalse(ClassUtils.isAssignable(Boolean.TYPE, Boolean.class, false));
assertFalse(ClassUtils.isAssignable(Boolean.TYPE, Object.class, false));
assertFalse(ClassUtils.isAssignable(Boolean.class, Boolean.TYPE, false));
assertTrue(ClassUtils.isAssignable(Boolean.class, Object.class, false));
assertTrue(ClassUtils.isAssignable(Boolean.TYPE, Boolean.TYPE, false));
assertTrue(ClassUtils.isAssignable(Boolean.class, Boolean.class, false));
}
public void test_isAssignable_Widening() throws Exception {
// test byte conversions
assertFalse("byte -> char", ClassUtils.isAssignable(Byte.TYPE, Character.TYPE));
assertTrue("byte -> byte", ClassUtils.isAssignable(Byte.TYPE, Byte.TYPE));
assertTrue("byte -> short", ClassUtils.isAssignable(Byte.TYPE, Short.TYPE));
assertTrue("byte -> int", ClassUtils.isAssignable(Byte.TYPE, Integer.TYPE));
assertTrue("byte -> long", ClassUtils.isAssignable(Byte.TYPE, Long.TYPE));
assertTrue("byte -> float", ClassUtils.isAssignable(Byte.TYPE, Float.TYPE));
assertTrue("byte -> double", ClassUtils.isAssignable(Byte.TYPE, Double.TYPE));
assertFalse("byte -> boolean", ClassUtils.isAssignable(Byte.TYPE, Boolean.TYPE));
// test short conversions
assertFalse("short -> char", ClassUtils.isAssignable(Short.TYPE, Character.TYPE));
assertFalse("short -> byte", ClassUtils.isAssignable(Short.TYPE, Byte.TYPE));
assertTrue("short -> short", ClassUtils.isAssignable(Short.TYPE, Short.TYPE));
assertTrue("short -> int", ClassUtils.isAssignable(Short.TYPE, Integer.TYPE));
assertTrue("short -> long", ClassUtils.isAssignable(Short.TYPE, Long.TYPE));
assertTrue("short -> float", ClassUtils.isAssignable(Short.TYPE, Float.TYPE));
assertTrue("short -> double", ClassUtils.isAssignable(Short.TYPE, Double.TYPE));
assertFalse("short -> boolean", ClassUtils.isAssignable(Short.TYPE, Boolean.TYPE));
// test char conversions
assertTrue("char -> char", ClassUtils.isAssignable(Character.TYPE, Character.TYPE));
assertFalse("char -> byte", ClassUtils.isAssignable(Character.TYPE, Byte.TYPE));
assertFalse("char -> short", ClassUtils.isAssignable(Character.TYPE, Short.TYPE));
assertTrue("char -> int", ClassUtils.isAssignable(Character.TYPE, Integer.TYPE));
assertTrue("char -> long", ClassUtils.isAssignable(Character.TYPE, Long.TYPE));
assertTrue("char -> float", ClassUtils.isAssignable(Character.TYPE, Float.TYPE));
assertTrue("char -> double", ClassUtils.isAssignable(Character.TYPE, Double.TYPE));
assertFalse("char -> boolean", ClassUtils.isAssignable(Character.TYPE, Boolean.TYPE));
// test int conversions
assertFalse("int -> char", ClassUtils.isAssignable(Integer.TYPE, Character.TYPE));
assertFalse("int -> byte", ClassUtils.isAssignable(Integer.TYPE, Byte.TYPE));
assertFalse("int -> short", ClassUtils.isAssignable(Integer.TYPE, Short.TYPE));
assertTrue("int -> int", ClassUtils.isAssignable(Integer.TYPE, Integer.TYPE));
assertTrue("int -> long", ClassUtils.isAssignable(Integer.TYPE, Long.TYPE));
assertTrue("int -> float", ClassUtils.isAssignable(Integer.TYPE, Float.TYPE));
assertTrue("int -> double", ClassUtils.isAssignable(Integer.TYPE, Double.TYPE));
assertFalse("int -> boolean", ClassUtils.isAssignable(Integer.TYPE, Boolean.TYPE));
// test long conversions
assertFalse("long -> char", ClassUtils.isAssignable(Long.TYPE, Character.TYPE));
assertFalse("long -> byte", ClassUtils.isAssignable(Long.TYPE, Byte.TYPE));
assertFalse("long -> short", ClassUtils.isAssignable(Long.TYPE, Short.TYPE));
assertFalse("long -> int", ClassUtils.isAssignable(Long.TYPE, Integer.TYPE));
assertTrue("long -> long", ClassUtils.isAssignable(Long.TYPE, Long.TYPE));
assertTrue("long -> float", ClassUtils.isAssignable(Long.TYPE, Float.TYPE));
assertTrue("long -> double", ClassUtils.isAssignable(Long.TYPE, Double.TYPE));
assertFalse("long -> boolean", ClassUtils.isAssignable(Long.TYPE, Boolean.TYPE));
// test float conversions
assertFalse("float -> char", ClassUtils.isAssignable(Float.TYPE, Character.TYPE));
assertFalse("float -> byte", ClassUtils.isAssignable(Float.TYPE, Byte.TYPE));
assertFalse("float -> short", ClassUtils.isAssignable(Float.TYPE, Short.TYPE));
assertFalse("float -> int", ClassUtils.isAssignable(Float.TYPE, Integer.TYPE));
assertFalse("float -> long", ClassUtils.isAssignable(Float.TYPE, Long.TYPE));
assertTrue("float -> float", ClassUtils.isAssignable(Float.TYPE, Float.TYPE));
assertTrue("float -> double", ClassUtils.isAssignable(Float.TYPE, Double.TYPE));
assertFalse("float -> boolean", ClassUtils.isAssignable(Float.TYPE, Boolean.TYPE));
// test double conversions
assertFalse("double -> char", ClassUtils.isAssignable(Double.TYPE, Character.TYPE));
assertFalse("double -> byte", ClassUtils.isAssignable(Double.TYPE, Byte.TYPE));
assertFalse("double -> short", ClassUtils.isAssignable(Double.TYPE, Short.TYPE));
assertFalse("double -> int", ClassUtils.isAssignable(Double.TYPE, Integer.TYPE));
assertFalse("double -> long", ClassUtils.isAssignable(Double.TYPE, Long.TYPE));
assertFalse("double -> float", ClassUtils.isAssignable(Double.TYPE, Float.TYPE));
assertTrue("double -> double", ClassUtils.isAssignable(Double.TYPE, Double.TYPE));
assertFalse("double -> boolean", ClassUtils.isAssignable(Double.TYPE, Boolean.TYPE));
// test boolean conversions
assertFalse("boolean -> char", ClassUtils.isAssignable(Boolean.TYPE, Character.TYPE));
assertFalse("boolean -> byte", ClassUtils.isAssignable(Boolean.TYPE, Byte.TYPE));
assertFalse("boolean -> short", ClassUtils.isAssignable(Boolean.TYPE, Short.TYPE));
assertFalse("boolean -> int", ClassUtils.isAssignable(Boolean.TYPE, Integer.TYPE));
assertFalse("boolean -> long", ClassUtils.isAssignable(Boolean.TYPE, Long.TYPE));
assertFalse("boolean -> float", ClassUtils.isAssignable(Boolean.TYPE, Float.TYPE));
assertFalse("boolean -> double", ClassUtils.isAssignable(Boolean.TYPE, Double.TYPE));
assertTrue("boolean -> boolean", ClassUtils.isAssignable(Boolean.TYPE, Boolean.TYPE));
}
public void test_isAssignable_DefaultUnboxing_Widening() throws Exception {
boolean autoboxing = SystemUtils.isJavaVersionAtLeast(1.5f);
// test byte conversions
assertFalse("byte -> char", ClassUtils.isAssignable(Byte.class, Character.TYPE));
assertEquals("byte -> byte", autoboxing, ClassUtils.isAssignable(Byte.class, Byte.TYPE));
assertEquals("byte -> short", autoboxing, ClassUtils.isAssignable(Byte.class, Short.TYPE));
assertEquals("byte -> int", autoboxing, ClassUtils.isAssignable(Byte.class, Integer.TYPE));
assertEquals("byte -> long", autoboxing, ClassUtils.isAssignable(Byte.class, Long.TYPE));
assertEquals("byte -> float", autoboxing, ClassUtils.isAssignable(Byte.class, Float.TYPE));
assertEquals("byte -> double", autoboxing, ClassUtils.isAssignable(Byte.class, Double.TYPE));
assertFalse("byte -> boolean", ClassUtils.isAssignable(Byte.class, Boolean.TYPE));
// test short conversions
assertFalse("short -> char", ClassUtils.isAssignable(Short.class, Character.TYPE));
assertFalse("short -> byte", ClassUtils.isAssignable(Short.class, Byte.TYPE));
assertEquals("short -> short", autoboxing, ClassUtils.isAssignable(Short.class, Short.TYPE));
assertEquals("short -> int", autoboxing, ClassUtils.isAssignable(Short.class, Integer.TYPE));
assertEquals("short -> long", autoboxing, ClassUtils.isAssignable(Short.class, Long.TYPE));
assertEquals("short -> float", autoboxing, ClassUtils.isAssignable(Short.class, Float.TYPE));
assertEquals("short -> double", autoboxing, ClassUtils.isAssignable(Short.class, Double.TYPE));
assertFalse("short -> boolean", ClassUtils.isAssignable(Short.class, Boolean.TYPE));
// test char conversions
assertEquals("char -> char", autoboxing, ClassUtils.isAssignable(Character.class, Character.TYPE));
assertFalse("char -> byte", ClassUtils.isAssignable(Character.class, Byte.TYPE));
assertFalse("char -> short", ClassUtils.isAssignable(Character.class, Short.TYPE));
assertEquals("char -> int", autoboxing, ClassUtils.isAssignable(Character.class, Integer.TYPE));
assertEquals("char -> long", autoboxing, ClassUtils.isAssignable(Character.class, Long.TYPE));
assertEquals("char -> float", autoboxing, ClassUtils.isAssignable(Character.class, Float.TYPE));
assertEquals("char -> double", autoboxing, ClassUtils.isAssignable(Character.class, Double.TYPE));
assertFalse("char -> boolean", ClassUtils.isAssignable(Character.class, Boolean.TYPE));
// test int conversions
assertFalse("int -> char", ClassUtils.isAssignable(Integer.class, Character.TYPE));
assertFalse("int -> byte", ClassUtils.isAssignable(Integer.class, Byte.TYPE));
assertFalse("int -> short", ClassUtils.isAssignable(Integer.class, Short.TYPE));
assertEquals("int -> int", autoboxing, ClassUtils.isAssignable(Integer.class, Integer.TYPE));
assertEquals("int -> long", autoboxing, ClassUtils.isAssignable(Integer.class, Long.TYPE));
assertEquals("int -> float", autoboxing, ClassUtils.isAssignable(Integer.class, Float.TYPE));
assertEquals("int -> double", autoboxing, ClassUtils.isAssignable(Integer.class, Double.TYPE));
assertFalse("int -> boolean", ClassUtils.isAssignable(Integer.class, Boolean.TYPE));
// test long conversions
assertFalse("long -> char", ClassUtils.isAssignable(Long.class, Character.TYPE));
assertFalse("long -> byte", ClassUtils.isAssignable(Long.class, Byte.TYPE));
assertFalse("long -> short", ClassUtils.isAssignable(Long.class, Short.TYPE));
assertFalse("long -> int", ClassUtils.isAssignable(Long.class, Integer.TYPE));
assertEquals("long -> long", autoboxing, ClassUtils.isAssignable(Long.class, Long.TYPE));
assertEquals("long -> float", autoboxing, ClassUtils.isAssignable(Long.class, Float.TYPE));
assertEquals("long -> double", autoboxing, ClassUtils.isAssignable(Long.class, Double.TYPE));
assertFalse("long -> boolean", ClassUtils.isAssignable(Long.class, Boolean.TYPE));
// test float conversions
assertFalse("float -> char", ClassUtils.isAssignable(Float.class, Character.TYPE));
assertFalse("float -> byte", ClassUtils.isAssignable(Float.class, Byte.TYPE));
assertFalse("float -> short", ClassUtils.isAssignable(Float.class, Short.TYPE));
assertFalse("float -> int", ClassUtils.isAssignable(Float.class, Integer.TYPE));
assertFalse("float -> long", ClassUtils.isAssignable(Float.class, Long.TYPE));
assertEquals("float -> float", autoboxing, ClassUtils.isAssignable(Float.class, Float.TYPE));
assertEquals("float -> double", autoboxing, ClassUtils.isAssignable(Float.class, Double.TYPE));
assertFalse("float -> boolean", ClassUtils.isAssignable(Float.class, Boolean.TYPE));
// test double conversions
assertFalse("double -> char", ClassUtils.isAssignable(Double.class, Character.TYPE));
assertFalse("double -> byte", ClassUtils.isAssignable(Double.class, Byte.TYPE));
assertFalse("double -> short", ClassUtils.isAssignable(Double.class, Short.TYPE));
assertFalse("double -> int", ClassUtils.isAssignable(Double.class, Integer.TYPE));
assertFalse("double -> long", ClassUtils.isAssignable(Double.class, Long.TYPE));
assertFalse("double -> float", ClassUtils.isAssignable(Double.class, Float.TYPE));
assertEquals("double -> double", autoboxing, ClassUtils.isAssignable(Double.class, Double.TYPE));
assertFalse("double -> boolean", ClassUtils.isAssignable(Double.class, Boolean.TYPE));
// test boolean conversions
assertFalse("boolean -> char", ClassUtils.isAssignable(Boolean.class, Character.TYPE));
assertFalse("boolean -> byte", ClassUtils.isAssignable(Boolean.class, Byte.TYPE));
assertFalse("boolean -> short", ClassUtils.isAssignable(Boolean.class, Short.TYPE));
assertFalse("boolean -> int", ClassUtils.isAssignable(Boolean.class, Integer.TYPE));
assertFalse("boolean -> long", ClassUtils.isAssignable(Boolean.class, Long.TYPE));
assertFalse("boolean -> float", ClassUtils.isAssignable(Boolean.class, Float.TYPE));
assertFalse("boolean -> double", ClassUtils.isAssignable(Boolean.class, Double.TYPE));
assertEquals("boolean -> boolean", autoboxing, ClassUtils.isAssignable(Boolean.class, Boolean.TYPE));
}
public void test_isAssignable_Unboxing_Widening() throws Exception {
// test byte conversions
assertFalse("byte -> char", ClassUtils.isAssignable(Byte.class, Character.TYPE, true));
assertTrue("byte -> byte", ClassUtils.isAssignable(Byte.class, Byte.TYPE, true));
assertTrue("byte -> short", ClassUtils.isAssignable(Byte.class, Short.TYPE, true));
assertTrue("byte -> int", ClassUtils.isAssignable(Byte.class, Integer.TYPE, true));
assertTrue("byte -> long", ClassUtils.isAssignable(Byte.class, Long.TYPE, true));
assertTrue("byte -> float", ClassUtils.isAssignable(Byte.class, Float.TYPE, true));
assertTrue("byte -> double", ClassUtils.isAssignable(Byte.class, Double.TYPE, true));
assertFalse("byte -> boolean", ClassUtils.isAssignable(Byte.class, Boolean.TYPE, true));
// test short conversions
assertFalse("short -> char", ClassUtils.isAssignable(Short.class, Character.TYPE, true));
assertFalse("short -> byte", ClassUtils.isAssignable(Short.class, Byte.TYPE, true));
assertTrue("short -> short", ClassUtils.isAssignable(Short.class, Short.TYPE, true));
assertTrue("short -> int", ClassUtils.isAssignable(Short.class, Integer.TYPE, true));
assertTrue("short -> long", ClassUtils.isAssignable(Short.class, Long.TYPE, true));
assertTrue("short -> float", ClassUtils.isAssignable(Short.class, Float.TYPE, true));
assertTrue("short -> double", ClassUtils.isAssignable(Short.class, Double.TYPE, true));
assertFalse("short -> boolean", ClassUtils.isAssignable(Short.class, Boolean.TYPE, true));
// test char conversions
assertTrue("char -> char", ClassUtils.isAssignable(Character.class, Character.TYPE, true));
assertFalse("char -> byte", ClassUtils.isAssignable(Character.class, Byte.TYPE, true));
assertFalse("char -> short", ClassUtils.isAssignable(Character.class, Short.TYPE, true));
assertTrue("char -> int", ClassUtils.isAssignable(Character.class, Integer.TYPE, true));
assertTrue("char -> long", ClassUtils.isAssignable(Character.class, Long.TYPE, true));
assertTrue("char -> float", ClassUtils.isAssignable(Character.class, Float.TYPE, true));
assertTrue("char -> double", ClassUtils.isAssignable(Character.class, Double.TYPE, true));
assertFalse("char -> boolean", ClassUtils.isAssignable(Character.class, Boolean.TYPE, true));
// test int conversions
assertFalse("int -> char", ClassUtils.isAssignable(Integer.class, Character.TYPE, true));
assertFalse("int -> byte", ClassUtils.isAssignable(Integer.class, Byte.TYPE, true));
assertFalse("int -> short", ClassUtils.isAssignable(Integer.class, Short.TYPE, true));
assertTrue("int -> int", ClassUtils.isAssignable(Integer.class, Integer.TYPE, true));
assertTrue("int -> long", ClassUtils.isAssignable(Integer.class, Long.TYPE, true));
assertTrue("int -> float", ClassUtils.isAssignable(Integer.class, Float.TYPE, true));
assertTrue("int -> double", ClassUtils.isAssignable(Integer.class, Double.TYPE, true));
assertFalse("int -> boolean", ClassUtils.isAssignable(Integer.class, Boolean.TYPE, true));
// test long conversions
assertFalse("long -> char", ClassUtils.isAssignable(Long.class, Character.TYPE, true));
assertFalse("long -> byte", ClassUtils.isAssignable(Long.class, Byte.TYPE, true));
assertFalse("long -> short", ClassUtils.isAssignable(Long.class, Short.TYPE, true));
assertFalse("long -> int", ClassUtils.isAssignable(Long.class, Integer.TYPE, true));
assertTrue("long -> long", ClassUtils.isAssignable(Long.class, Long.TYPE, true));
assertTrue("long -> float", ClassUtils.isAssignable(Long.class, Float.TYPE, true));
assertTrue("long -> double", ClassUtils.isAssignable(Long.class, Double.TYPE, true));
assertFalse("long -> boolean", ClassUtils.isAssignable(Long.class, Boolean.TYPE, true));
// test float conversions
assertFalse("float -> char", ClassUtils.isAssignable(Float.class, Character.TYPE, true));
assertFalse("float -> byte", ClassUtils.isAssignable(Float.class, Byte.TYPE, true));
assertFalse("float -> short", ClassUtils.isAssignable(Float.class, Short.TYPE, true));
assertFalse("float -> int", ClassUtils.isAssignable(Float.class, Integer.TYPE, true));
assertFalse("float -> long", ClassUtils.isAssignable(Float.class, Long.TYPE, true));
assertTrue("float -> float", ClassUtils.isAssignable(Float.class, Float.TYPE, true));
assertTrue("float -> double", ClassUtils.isAssignable(Float.class, Double.TYPE, true));
assertFalse("float -> boolean", ClassUtils.isAssignable(Float.class, Boolean.TYPE, true));
// test double conversions
assertFalse("double -> char", ClassUtils.isAssignable(Double.class, Character.TYPE, true));
assertFalse("double -> byte", ClassUtils.isAssignable(Double.class, Byte.TYPE, true));
assertFalse("double -> short", ClassUtils.isAssignable(Double.class, Short.TYPE, true));
assertFalse("double -> int", ClassUtils.isAssignable(Double.class, Integer.TYPE, true));
assertFalse("double -> long", ClassUtils.isAssignable(Double.class, Long.TYPE, true));
assertFalse("double -> float", ClassUtils.isAssignable(Double.class, Float.TYPE, true));
assertTrue("double -> double", ClassUtils.isAssignable(Double.class, Double.TYPE, true));
assertFalse("double -> boolean", ClassUtils.isAssignable(Double.class, Boolean.TYPE, true));
// test boolean conversions
assertFalse("boolean -> char", ClassUtils.isAssignable(Boolean.class, Character.TYPE, true));
assertFalse("boolean -> byte", ClassUtils.isAssignable(Boolean.class, Byte.TYPE, true));
assertFalse("boolean -> short", ClassUtils.isAssignable(Boolean.class, Short.TYPE, true));
assertFalse("boolean -> int", ClassUtils.isAssignable(Boolean.class, Integer.TYPE, true));
assertFalse("boolean -> long", ClassUtils.isAssignable(Boolean.class, Long.TYPE, true));
assertFalse("boolean -> float", ClassUtils.isAssignable(Boolean.class, Float.TYPE, true));
assertFalse("boolean -> double", ClassUtils.isAssignable(Boolean.class, Double.TYPE, true));
assertTrue("boolean -> boolean", ClassUtils.isAssignable(Boolean.class, Boolean.TYPE, true));
}
public void testPrimitiveToWrapper() {
// test primitive classes
assertEquals("boolean -> Boolean.class",
Boolean.class, ClassUtils.primitiveToWrapper(Boolean.TYPE));
assertEquals("byte -> Byte.class",
Byte.class, ClassUtils.primitiveToWrapper(Byte.TYPE));
assertEquals("char -> Character.class",
Character.class, ClassUtils.primitiveToWrapper(Character.TYPE));
assertEquals("short -> Short.class",
Short.class, ClassUtils.primitiveToWrapper(Short.TYPE));
assertEquals("int -> Integer.class",
Integer.class, ClassUtils.primitiveToWrapper(Integer.TYPE));
assertEquals("long -> Long.class",
Long.class, ClassUtils.primitiveToWrapper(Long.TYPE));
assertEquals("double -> Double.class",
Double.class, ClassUtils.primitiveToWrapper(Double.TYPE));
assertEquals("float -> Float.class",
Float.class, ClassUtils.primitiveToWrapper(Float.TYPE));
// test a few other classes
assertEquals("String.class -> String.class",
String.class, ClassUtils.primitiveToWrapper(String.class));
assertEquals("ClassUtils.class -> ClassUtils.class",
org.apache.commons.lang3.ClassUtils.class,
ClassUtils.primitiveToWrapper(org.apache.commons.lang3.ClassUtils.class));
assertEquals("Void.TYPE -> Void.TYPE",
Void.TYPE, ClassUtils.primitiveToWrapper(Void.TYPE));
// test null
assertNull("null -> null",
ClassUtils.primitiveToWrapper(null));
}
public void testPrimitivesToWrappers() {
// test null
assertNull("null -> null",
ClassUtils.primitivesToWrappers(null));
// test empty array
assertEquals("empty -> empty",
ArrayUtils.EMPTY_CLASS_ARRAY, ClassUtils.primitivesToWrappers(ArrayUtils.EMPTY_CLASS_ARRAY));
// test an array of various classes
final Class<?>[] primitives = new Class[] {
Boolean.TYPE, Byte.TYPE, Character.TYPE, Short.TYPE,
Integer.TYPE, Long.TYPE, Double.TYPE, Float.TYPE,
String.class, ClassUtils.class
};
Class<?>[] wrappers= ClassUtils.primitivesToWrappers(primitives);
for (int i=0; i < primitives.length; i++) {
// test each returned wrapper
Class<?> primitive = primitives[i];
Class<?> expectedWrapper = ClassUtils.primitiveToWrapper(primitive);
assertEquals(primitive + " -> " + expectedWrapper, expectedWrapper, wrappers[i]);
}
// test an array of no primitive classes
final Class<?>[] noPrimitives = new Class[] {
String.class, ClassUtils.class, Void.TYPE
};
// This used to return the exact same array, but no longer does.
assertNotSame("unmodified", noPrimitives, ClassUtils.primitivesToWrappers(noPrimitives));
}
public void testWrapperToPrimitive() {
// an array with classes to convert
final Class<?>[] primitives = {
Boolean.TYPE, Byte.TYPE, Character.TYPE, Short.TYPE,
Integer.TYPE, Long.TYPE, Float.TYPE, Double.TYPE
};
for (int i = 0; i < primitives.length; i++) {
Class<?> wrapperCls = ClassUtils.primitiveToWrapper(primitives[i]);
assertFalse("Still primitive", wrapperCls.isPrimitive());
assertEquals(wrapperCls + " -> " + primitives[i], primitives[i],
ClassUtils.wrapperToPrimitive(wrapperCls));
}
}
public void testWrapperToPrimitiveNoWrapper() {
assertNull("Wrong result for non wrapper class", ClassUtils.wrapperToPrimitive(String.class));
}
public void testWrapperToPrimitiveNull() {
assertNull("Wrong result for null class", ClassUtils.wrapperToPrimitive(null));
}
public void testWrappersToPrimitives() {
// an array with classes to test
final Class<?>[] classes = {
Boolean.class, Byte.class, Character.class, Short.class,
Integer.class, Long.class, Float.class, Double.class,
String.class, ClassUtils.class, null
};
Class<?>[] primitives = ClassUtils.wrappersToPrimitives(classes);
// now test the result
assertEquals("Wrong length of result array", classes.length, primitives.length);
for (int i = 0; i < classes.length; i++) {
Class<?> expectedPrimitive = ClassUtils.wrapperToPrimitive(classes[i]);
assertEquals(classes[i] + " -> " + expectedPrimitive, expectedPrimitive,
primitives[i]);
}
}
public void testWrappersToPrimitivesNull() {
assertNull("Wrong result for null input", ClassUtils.wrappersToPrimitives(null));
}
public void testWrappersToPrimitivesEmpty() {
Class<?>[] empty = new Class[0];
assertEquals("Wrong result for empty input", empty, ClassUtils.wrappersToPrimitives(empty));
}
public void testGetClassClassNotFound() throws Exception {
assertGetClassThrowsClassNotFound( "bool" );
assertGetClassThrowsClassNotFound( "bool[]" );
assertGetClassThrowsClassNotFound( "integer[]" );
}
public void testGetClassInvalidArguments() throws Exception {
assertGetClassThrowsNullPointerException( null );
assertGetClassThrowsClassNotFound( "[][][]" );
assertGetClassThrowsClassNotFound( "[[]" );
assertGetClassThrowsClassNotFound( "[" );
assertGetClassThrowsClassNotFound( "java.lang.String][" );
assertGetClassThrowsClassNotFound( ".hello.world" );
assertGetClassThrowsClassNotFound( "hello..world" );
}
public void testWithInterleavingWhitespace() throws ClassNotFoundException {
assertEquals( int[].class, ClassUtils.getClass( " int [ ] " ) );
assertEquals( long[].class, ClassUtils.getClass( "\rlong\t[\n]\r" ) );
assertEquals( short[].class, ClassUtils.getClass( "\tshort \t\t[]" ) );
assertEquals( byte[].class, ClassUtils.getClass( "byte[\t\t\n\r] " ) );
}
public void testGetClassByNormalNameArrays() throws ClassNotFoundException {
assertEquals( int[].class, ClassUtils.getClass( "int[]" ) );
assertEquals( long[].class, ClassUtils.getClass( "long[]" ) );
assertEquals( short[].class, ClassUtils.getClass( "short[]" ) );
assertEquals( byte[].class, ClassUtils.getClass( "byte[]" ) );
assertEquals( char[].class, ClassUtils.getClass( "char[]" ) );
assertEquals( float[].class, ClassUtils.getClass( "float[]" ) );
assertEquals( double[].class, ClassUtils.getClass( "double[]" ) );
assertEquals( boolean[].class, ClassUtils.getClass( "boolean[]" ) );
assertEquals( String[].class, ClassUtils.getClass( "java.lang.String[]" ) );
}
public void testGetClassByNormalNameArrays2D() throws ClassNotFoundException {
assertEquals( int[][].class, ClassUtils.getClass( "int[][]" ) );
assertEquals( long[][].class, ClassUtils.getClass( "long[][]" ) );
assertEquals( short[][].class, ClassUtils.getClass( "short[][]" ) );
assertEquals( byte[][].class, ClassUtils.getClass( "byte[][]" ) );
assertEquals( char[][].class, ClassUtils.getClass( "char[][]" ) );
assertEquals( float[][].class, ClassUtils.getClass( "float[][]" ) );
assertEquals( double[][].class, ClassUtils.getClass( "double[][]" ) );
assertEquals( boolean[][].class, ClassUtils.getClass( "boolean[][]" ) );
assertEquals( String[][].class, ClassUtils.getClass( "java.lang.String[][]" ) );
}
public void testGetClassWithArrayClasses2D() throws Exception {
assertGetClassReturnsClass( String[][].class );
assertGetClassReturnsClass( int[][].class );
assertGetClassReturnsClass( long[][].class );
assertGetClassReturnsClass( short[][].class );
assertGetClassReturnsClass( byte[][].class );
assertGetClassReturnsClass( char[][].class );
assertGetClassReturnsClass( float[][].class );
assertGetClassReturnsClass( double[][].class );
assertGetClassReturnsClass( boolean[][].class );
}
public void testGetClassWithArrayClasses() throws Exception {
assertGetClassReturnsClass( String[].class );
assertGetClassReturnsClass( int[].class );
assertGetClassReturnsClass( long[].class );
assertGetClassReturnsClass( short[].class );
assertGetClassReturnsClass( byte[].class );
assertGetClassReturnsClass( char[].class );
assertGetClassReturnsClass( float[].class );
assertGetClassReturnsClass( double[].class );
assertGetClassReturnsClass( boolean[].class );
}
public void testGetClassRawPrimitives() throws ClassNotFoundException {
assertEquals( int.class, ClassUtils.getClass( "int" ) );
assertEquals( long.class, ClassUtils.getClass( "long" ) );
assertEquals( short.class, ClassUtils.getClass( "short" ) );
assertEquals( byte.class, ClassUtils.getClass( "byte" ) );
assertEquals( char.class, ClassUtils.getClass( "char" ) );
assertEquals( float.class, ClassUtils.getClass( "float" ) );
assertEquals( double.class, ClassUtils.getClass( "double" ) );
assertEquals( boolean.class, ClassUtils.getClass( "boolean" ) );
}
private void assertGetClassReturnsClass( Class<?> c ) throws Exception {
assertEquals( c, ClassUtils.getClass( c.getName() ) );
}
private void assertGetClassThrowsException( String className, Class<?> exceptionType ) throws Exception {
try {
ClassUtils.getClass( className );
fail( "ClassUtils.getClass() should fail with an exception of type " + exceptionType.getName() + " when given class name \"" + className + "\"." );
}
catch( Exception e ) {
assertTrue( exceptionType.isAssignableFrom( e.getClass() ) );
}
}
private void assertGetClassThrowsNullPointerException( String className ) throws Exception {
assertGetClassThrowsException( className, NullPointerException.class );
}
private void assertGetClassThrowsClassNotFound( String className ) throws Exception {
assertGetClassThrowsException( className, ClassNotFoundException.class );
}
// Show the Java bug: http://bugs.sun.com/bugdatabase/view_bug.do?bug_id=4071957
// We may have to delete this if a JDK fixes the bug.
public void testShowJavaBug() throws Exception {
// Tests with Collections$UnmodifiableSet
Set<?> set = Collections.unmodifiableSet(new HashSet<Object>());
Method isEmptyMethod = set.getClass().getMethod("isEmpty", new Class[0]);
try {
isEmptyMethod.invoke(set, new Object[0]);
fail("Failed to throw IllegalAccessException as expected");
} catch(IllegalAccessException iae) {
// expected
}
}
public void testGetPublicMethod() throws Exception {
// Tests with Collections$UnmodifiableSet
Set<?> set = Collections.unmodifiableSet(new HashSet<Object>());
Method isEmptyMethod = ClassUtils.getPublicMethod(set.getClass(), "isEmpty", new Class[0]);
assertTrue(Modifier.isPublic(isEmptyMethod.getDeclaringClass().getModifiers()));
try {
isEmptyMethod.invoke(set, new Object[0]);
} catch(java.lang.IllegalAccessException iae) {
fail("Should not have thrown IllegalAccessException");
}
// Tests with a public Class
Method toStringMethod = ClassUtils.getPublicMethod(Object.class, "toString", new Class[0]);
assertEquals(Object.class.getMethod("toString", new Class[0]), toStringMethod);
}
public void testToClass_object() {
assertNull(ClassUtils.toClass(null));
assertSame(ArrayUtils.EMPTY_CLASS_ARRAY, ClassUtils.toClass(ArrayUtils.EMPTY_OBJECT_ARRAY));
assertTrue(Arrays.equals(new Class[] { String.class, Integer.class, Double.class },
ClassUtils.toClass(new Object[] { "Test", 1, 99d })));
assertTrue(Arrays.equals(new Class[] { String.class, null, Double.class },
ClassUtils.toClass(new Object[] { "Test", null, 99d })));
}
public void test_getShortCanonicalName_Object() {
assertEquals("<null>", ClassUtils.getShortCanonicalName(null, "<null>"));
assertEquals("ClassUtils", ClassUtils.getShortCanonicalName(new ClassUtils(), "<null>"));
assertEquals("ClassUtils[]", ClassUtils.getShortCanonicalName(new ClassUtils[0], "<null>"));
assertEquals("ClassUtils[][]", ClassUtils.getShortCanonicalName(new ClassUtils[0][0], "<null>"));
assertEquals("int[]", ClassUtils.getShortCanonicalName(new int[0], "<null>"));
assertEquals("int[][]", ClassUtils.getShortCanonicalName(new int[0][0], "<null>"));
}
public void test_getShortCanonicalName_Class() {
assertEquals("ClassUtils", ClassUtils.getShortCanonicalName(ClassUtils.class));
assertEquals("ClassUtils[]", ClassUtils.getShortCanonicalName(ClassUtils[].class));
assertEquals("ClassUtils[][]", ClassUtils.getShortCanonicalName(ClassUtils[][].class));
assertEquals("int[]", ClassUtils.getShortCanonicalName(int[].class));
assertEquals("int[][]", ClassUtils.getShortCanonicalName(int[][].class));
}
public void test_getShortCanonicalName_String() {
assertEquals("ClassUtils", ClassUtils.getShortCanonicalName("org.apache.commons.lang3.ClassUtils"));
assertEquals("ClassUtils[]", ClassUtils.getShortCanonicalName("[Lorg.apache.commons.lang3.ClassUtils;"));
assertEquals("ClassUtils[][]", ClassUtils.getShortCanonicalName("[[Lorg.apache.commons.lang3.ClassUtils;"));
assertEquals("ClassUtils[]", ClassUtils.getShortCanonicalName("org.apache.commons.lang3.ClassUtils[]"));
assertEquals("ClassUtils[][]", ClassUtils.getShortCanonicalName("org.apache.commons.lang3.ClassUtils[][]"));
assertEquals("int[]", ClassUtils.getShortCanonicalName("[I"));
assertEquals("int[][]", ClassUtils.getShortCanonicalName("[[I"));
assertEquals("int[]", ClassUtils.getShortCanonicalName("int[]"));
assertEquals("int[][]", ClassUtils.getShortCanonicalName("int[][]"));
}
public void test_getPackageCanonicalName_Object() {
assertEquals("<null>", ClassUtils.getPackageCanonicalName(null, "<null>"));
assertEquals("org.apache.commons.lang3", ClassUtils.getPackageCanonicalName(new ClassUtils(), "<null>"));
assertEquals("org.apache.commons.lang3", ClassUtils.getPackageCanonicalName(new ClassUtils[0], "<null>"));
assertEquals("org.apache.commons.lang3", ClassUtils.getPackageCanonicalName(new ClassUtils[0][0], "<null>"));
assertEquals("", ClassUtils.getPackageCanonicalName(new int[0], "<null>"));
assertEquals("", ClassUtils.getPackageCanonicalName(new int[0][0], "<null>"));
}
public void test_getPackageCanonicalName_Class() {
assertEquals("org.apache.commons.lang3", ClassUtils.getPackageCanonicalName(ClassUtils.class));
assertEquals("org.apache.commons.lang3", ClassUtils.getPackageCanonicalName(ClassUtils[].class));
assertEquals("org.apache.commons.lang3", ClassUtils.getPackageCanonicalName(ClassUtils[][].class));
assertEquals("", ClassUtils.getPackageCanonicalName(int[].class));
assertEquals("", ClassUtils.getPackageCanonicalName(int[][].class));
}
public void test_getPackageCanonicalName_String() {
assertEquals("org.apache.commons.lang3",
ClassUtils.getPackageCanonicalName("org.apache.commons.lang3.ClassUtils"));
assertEquals("org.apache.commons.lang3",
ClassUtils.getPackageCanonicalName("[Lorg.apache.commons.lang3.ClassUtils;"));
assertEquals("org.apache.commons.lang3",
ClassUtils.getPackageCanonicalName("[[Lorg.apache.commons.lang3.ClassUtils;"));
assertEquals("org.apache.commons.lang3",
ClassUtils.getPackageCanonicalName("org.apache.commons.lang3.ClassUtils[]"));
assertEquals("org.apache.commons.lang3",
ClassUtils.getPackageCanonicalName("org.apache.commons.lang3.ClassUtils[][]"));
assertEquals("", ClassUtils.getPackageCanonicalName("[I"));
assertEquals("", ClassUtils.getPackageCanonicalName("[[I"));
assertEquals("", ClassUtils.getPackageCanonicalName("int[]"));
assertEquals("", ClassUtils.getPackageCanonicalName("int[][]"));
}
} | // You are a professional Java test case writer, please create a test case named `testToClass_object` for the issue `Lang-LANG-587`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Lang-LANG-587
//
// ## Issue-Title:
// ClassUtils.toClass(Object[]) throws NPE on null array element
//
// ## Issue-Description:
//
// see summary
//
//
//
//
//
public void testToClass_object() {
| 911 | 33 | 901 | src/test/java/org/apache/commons/lang3/ClassUtilsTest.java | src/test/java | ```markdown
## Issue-ID: Lang-LANG-587
## Issue-Title:
ClassUtils.toClass(Object[]) throws NPE on null array element
## Issue-Description:
see summary
```
You are a professional Java test case writer, please create a test case named `testToClass_object` for the issue `Lang-LANG-587`, utilizing the provided issue report information and the following function signature.
```java
public void testToClass_object() {
```
| 901 | [
"org.apache.commons.lang3.ClassUtils"
] | 88f5adf757687983e9032f2aa61d98789baf908e410603fc95204a78c3fcc067 | public void testToClass_object() | // You are a professional Java test case writer, please create a test case named `testToClass_object` for the issue `Lang-LANG-587`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Lang-LANG-587
//
// ## Issue-Title:
// ClassUtils.toClass(Object[]) throws NPE on null array element
//
// ## Issue-Description:
//
// see summary
//
//
//
//
//
| Lang | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.lang3;
import java.lang.reflect.Constructor;
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import junit.framework.TestCase;
/**
* Unit tests {@link org.apache.commons.lang3.ClassUtils}.
*
* @author Apache Software Foundation
* @author Gary D. Gregory
* @author Tomasz Blachowicz
* @version $Id$
*/
public class ClassUtilsTest extends TestCase {
public ClassUtilsTest(String name) {
super(name);
}
private static class Inner {
}
//-----------------------------------------------------------------------
public void testConstructor() {
assertNotNull(new ClassUtils());
Constructor<?>[] cons = ClassUtils.class.getDeclaredConstructors();
assertEquals(1, cons.length);
assertEquals(true, Modifier.isPublic(cons[0].getModifiers()));
assertEquals(true, Modifier.isPublic(ClassUtils.class.getModifiers()));
assertEquals(false, Modifier.isFinal(ClassUtils.class.getModifiers()));
}
// -------------------------------------------------------------------------
public void test_getShortClassName_Object() {
assertEquals("ClassUtils", ClassUtils.getShortClassName(new ClassUtils(), "<null>"));
assertEquals("ClassUtilsTest.Inner", ClassUtils.getShortClassName(new Inner(), "<null>"));
assertEquals("String", ClassUtils.getShortClassName("hello", "<null>"));
assertEquals("<null>", ClassUtils.getShortClassName(null, "<null>"));
}
public void test_getShortClassName_Class() {
assertEquals("ClassUtils", ClassUtils.getShortClassName(ClassUtils.class));
assertEquals("Map.Entry", ClassUtils.getShortClassName(Map.Entry.class));
assertEquals("", ClassUtils.getShortClassName((Class<?>) null));
// LANG-535
assertEquals("String[]", ClassUtils.getShortClassName(String[].class));
assertEquals("Map.Entry[]", ClassUtils.getShortClassName(Map.Entry[].class));
// Primitives
assertEquals("boolean", ClassUtils.getShortClassName(boolean.class));
assertEquals("byte", ClassUtils.getShortClassName(byte.class));
assertEquals("char", ClassUtils.getShortClassName(char.class));
assertEquals("short", ClassUtils.getShortClassName(short.class));
assertEquals("int", ClassUtils.getShortClassName(int.class));
assertEquals("long", ClassUtils.getShortClassName(long.class));
assertEquals("float", ClassUtils.getShortClassName(float.class));
assertEquals("double", ClassUtils.getShortClassName(double.class));
// Primitive Arrays
assertEquals("boolean[]", ClassUtils.getShortClassName(boolean[].class));
assertEquals("byte[]", ClassUtils.getShortClassName(byte[].class));
assertEquals("char[]", ClassUtils.getShortClassName(char[].class));
assertEquals("short[]", ClassUtils.getShortClassName(short[].class));
assertEquals("int[]", ClassUtils.getShortClassName(int[].class));
assertEquals("long[]", ClassUtils.getShortClassName(long[].class));
assertEquals("float[]", ClassUtils.getShortClassName(float[].class));
assertEquals("double[]", ClassUtils.getShortClassName(double[].class));
// Arrays of arrays of ...
assertEquals("String[][]", ClassUtils.getShortClassName(String[][].class));
assertEquals("String[][][]", ClassUtils.getShortClassName(String[][][].class));
assertEquals("String[][][][]", ClassUtils.getShortClassName(String[][][][].class));
}
public void test_getShortClassName_String() {
assertEquals("ClassUtils", ClassUtils.getShortClassName(ClassUtils.class.getName()));
assertEquals("Map.Entry", ClassUtils.getShortClassName(Map.Entry.class.getName()));
assertEquals("", ClassUtils.getShortClassName((String) null));
assertEquals("", ClassUtils.getShortClassName(""));
}
// -------------------------------------------------------------------------
public void test_getPackageName_Object() {
assertEquals("org.apache.commons.lang3", ClassUtils.getPackageName(new ClassUtils(), "<null>"));
assertEquals("org.apache.commons.lang3", ClassUtils.getPackageName(new Inner(), "<null>"));
assertEquals("<null>", ClassUtils.getPackageName(null, "<null>"));
}
public void test_getPackageName_Class() {
assertEquals("java.lang", ClassUtils.getPackageName(String.class));
assertEquals("java.util", ClassUtils.getPackageName(Map.Entry.class));
assertEquals("", ClassUtils.getPackageName((Class<?>)null));
// LANG-535
assertEquals("java.lang", ClassUtils.getPackageName(String[].class));
// Primitive Arrays
assertEquals("", ClassUtils.getPackageName(boolean[].class));
assertEquals("", ClassUtils.getPackageName(byte[].class));
assertEquals("", ClassUtils.getPackageName(char[].class));
assertEquals("", ClassUtils.getPackageName(short[].class));
assertEquals("", ClassUtils.getPackageName(int[].class));
assertEquals("", ClassUtils.getPackageName(long[].class));
assertEquals("", ClassUtils.getPackageName(float[].class));
assertEquals("", ClassUtils.getPackageName(double[].class));
// Arrays of arrays of ...
assertEquals("java.lang", ClassUtils.getPackageName(String[][].class));
assertEquals("java.lang", ClassUtils.getPackageName(String[][][].class));
assertEquals("java.lang", ClassUtils.getPackageName(String[][][][].class));
}
public void test_getPackageName_String() {
assertEquals("org.apache.commons.lang3", ClassUtils.getPackageName(ClassUtils.class.getName()));
assertEquals("java.util", ClassUtils.getPackageName(Map.Entry.class.getName()));
assertEquals("", ClassUtils.getPackageName((String)null));
assertEquals("", ClassUtils.getPackageName(""));
}
// -------------------------------------------------------------------------
public void test_getAllSuperclasses_Class() {
List<?> list = ClassUtils.getAllSuperclasses(CY.class);
assertEquals(2, list.size());
assertEquals(CX.class, list.get(0));
assertEquals(Object.class, list.get(1));
assertEquals(null, ClassUtils.getAllSuperclasses(null));
}
public void test_getAllInterfaces_Class() {
List<?> list = ClassUtils.getAllInterfaces(CY.class);
assertEquals(6, list.size());
assertEquals(IB.class, list.get(0));
assertEquals(IC.class, list.get(1));
assertEquals(ID.class, list.get(2));
assertEquals(IE.class, list.get(3));
assertEquals(IF.class, list.get(4));
assertEquals(IA.class, list.get(5));
assertEquals(null, ClassUtils.getAllInterfaces(null));
}
private static interface IA {
}
private static interface IB {
}
private static interface IC extends ID, IE {
}
private static interface ID {
}
private static interface IE extends IF {
}
private static interface IF {
}
private static class CX implements IB, IA, IE {
}
private static class CY extends CX implements IB, IC {
}
// -------------------------------------------------------------------------
public void test_convertClassNamesToClasses_List() {
List<String> list = new ArrayList<String>();
List<Class<?>> result = ClassUtils.convertClassNamesToClasses(list);
assertEquals(0, result.size());
list.add("java.lang.String");
list.add("java.lang.xxx");
list.add("java.lang.Object");
result = ClassUtils.convertClassNamesToClasses(list);
assertEquals(3, result.size());
assertEquals(String.class, result.get(0));
assertEquals(null, result.get(1));
assertEquals(Object.class, result.get(2));
@SuppressWarnings("unchecked") // test what happens when non-gneric code adds wrong type of element
List<Object> olist = (List<Object>)(List<?>)list;
olist.add(new Object());
try {
ClassUtils.convertClassNamesToClasses(list);
fail("Should not have been able to convert list");
} catch (ClassCastException expected) {}
assertEquals(null, ClassUtils.convertClassNamesToClasses(null));
}
public void test_convertClassesToClassNames_List() {
List<Class<?>> list = new ArrayList<Class<?>>();
List<String> result = ClassUtils.convertClassesToClassNames(list);
assertEquals(0, result.size());
list.add(String.class);
list.add(null);
list.add(Object.class);
result = ClassUtils.convertClassesToClassNames(list);
assertEquals(3, result.size());
assertEquals("java.lang.String", result.get(0));
assertEquals(null, result.get(1));
assertEquals("java.lang.Object", result.get(2));
@SuppressWarnings("unchecked") // test what happens when non-gneric code adds wrong type of element
List<Object> olist = (List<Object>)(List<?>)list;
olist.add(new Object());
try {
ClassUtils.convertClassesToClassNames(list);
fail("Should not have been able to convert list");
} catch (ClassCastException expected) {}
assertEquals(null, ClassUtils.convertClassesToClassNames(null));
}
// -------------------------------------------------------------------------
public void test_isInnerClass_Class() {
assertEquals(true, ClassUtils.isInnerClass(Inner.class));
assertEquals(true, ClassUtils.isInnerClass(Map.Entry.class));
assertEquals(true, ClassUtils.isInnerClass(new Cloneable() {
}.getClass()));
assertEquals(false, ClassUtils.isInnerClass(this.getClass()));
assertEquals(false, ClassUtils.isInnerClass(String.class));
assertEquals(false, ClassUtils.isInnerClass(null));
}
// -------------------------------------------------------------------------
public void test_isAssignable_ClassArray_ClassArray() throws Exception {
Class<?>[] array2 = new Class[] {Object.class, Object.class};
Class<?>[] array1 = new Class[] {Object.class};
Class<?>[] array1s = new Class[] {String.class};
Class<?>[] array0 = new Class[] {};
Class<?>[] arrayPrimitives = { Integer.TYPE, Boolean.TYPE };
Class<?>[] arrayWrappers = { Integer.class, Boolean.class };
assertFalse(ClassUtils.isAssignable(array1, array2));
assertFalse(ClassUtils.isAssignable(null, array2));
assertTrue(ClassUtils.isAssignable(null, array0));
assertTrue(ClassUtils.isAssignable(array0, array0));
assertTrue(ClassUtils.isAssignable(array0, null));
assertTrue(ClassUtils.isAssignable((Class[]) null, (Class[]) null));
assertFalse(ClassUtils.isAssignable(array1, array1s));
assertTrue(ClassUtils.isAssignable(array1s, array1s));
assertTrue(ClassUtils.isAssignable(array1s, array1));
boolean autoboxing = SystemUtils.isJavaVersionAtLeast(1.5f);
assertEquals(autoboxing, ClassUtils.isAssignable(arrayPrimitives, arrayWrappers));
assertEquals(autoboxing, ClassUtils.isAssignable(arrayWrappers, arrayPrimitives));
assertFalse(ClassUtils.isAssignable(arrayPrimitives, array1));
assertFalse(ClassUtils.isAssignable(arrayWrappers, array1));
assertEquals(autoboxing, ClassUtils.isAssignable(arrayPrimitives, array2));
assertTrue(ClassUtils.isAssignable(arrayWrappers, array2));
}
public void test_isAssignable_ClassArray_ClassArray_Autoboxing() throws Exception {
Class<?>[] array2 = new Class[] {Object.class, Object.class};
Class<?>[] array1 = new Class[] {Object.class};
Class<?>[] array1s = new Class[] {String.class};
Class<?>[] array0 = new Class[] {};
Class<?>[] arrayPrimitives = { Integer.TYPE, Boolean.TYPE };
Class<?>[] arrayWrappers = { Integer.class, Boolean.class };
assertFalse(ClassUtils.isAssignable(array1, array2, true));
assertFalse(ClassUtils.isAssignable(null, array2, true));
assertTrue(ClassUtils.isAssignable(null, array0, true));
assertTrue(ClassUtils.isAssignable(array0, array0, true));
assertTrue(ClassUtils.isAssignable(array0, null, true));
assertTrue(ClassUtils.isAssignable((Class[]) null, (Class[]) null, true));
assertFalse(ClassUtils.isAssignable(array1, array1s, true));
assertTrue(ClassUtils.isAssignable(array1s, array1s, true));
assertTrue(ClassUtils.isAssignable(array1s, array1, true));
assertTrue(ClassUtils.isAssignable(arrayPrimitives, arrayWrappers, true));
assertTrue(ClassUtils.isAssignable(arrayWrappers, arrayPrimitives, true));
assertFalse(ClassUtils.isAssignable(arrayPrimitives, array1, true));
assertFalse(ClassUtils.isAssignable(arrayWrappers, array1, true));
assertTrue(ClassUtils.isAssignable(arrayPrimitives, array2, true));
assertTrue(ClassUtils.isAssignable(arrayWrappers, array2, true));
}
public void test_isAssignable_ClassArray_ClassArray_NoAutoboxing() throws Exception {
Class<?>[] array2 = new Class[] {Object.class, Object.class};
Class<?>[] array1 = new Class[] {Object.class};
Class<?>[] array1s = new Class[] {String.class};
Class<?>[] array0 = new Class[] {};
Class<?>[] arrayPrimitives = { Integer.TYPE, Boolean.TYPE };
Class<?>[] arrayWrappers = { Integer.class, Boolean.class };
assertFalse(ClassUtils.isAssignable(array1, array2, false));
assertFalse(ClassUtils.isAssignable(null, array2, false));
assertTrue(ClassUtils.isAssignable(null, array0, false));
assertTrue(ClassUtils.isAssignable(array0, array0, false));
assertTrue(ClassUtils.isAssignable(array0, null, false));
assertTrue(ClassUtils.isAssignable((Class[]) null, (Class[]) null, false));
assertFalse(ClassUtils.isAssignable(array1, array1s, false));
assertTrue(ClassUtils.isAssignable(array1s, array1s, false));
assertTrue(ClassUtils.isAssignable(array1s, array1, false));
assertFalse(ClassUtils.isAssignable(arrayPrimitives, arrayWrappers, false));
assertFalse(ClassUtils.isAssignable(arrayWrappers, arrayPrimitives, false));
assertFalse(ClassUtils.isAssignable(arrayPrimitives, array1, false));
assertFalse(ClassUtils.isAssignable(arrayWrappers, array1, false));
assertTrue(ClassUtils.isAssignable(arrayWrappers, array2, false));
assertFalse(ClassUtils.isAssignable(arrayPrimitives, array2, false));
}
public void test_isAssignable() throws Exception {
assertFalse(ClassUtils.isAssignable((Class<?>) null, null));
assertFalse(ClassUtils.isAssignable(String.class, null));
assertTrue(ClassUtils.isAssignable(null, Object.class));
assertTrue(ClassUtils.isAssignable(null, Integer.class));
assertFalse(ClassUtils.isAssignable(null, Integer.TYPE));
assertTrue(ClassUtils.isAssignable(String.class, Object.class));
assertTrue(ClassUtils.isAssignable(String.class, String.class));
assertFalse(ClassUtils.isAssignable(Object.class, String.class));
boolean autoboxing = SystemUtils.isJavaVersionAtLeast(1.5f);
assertEquals(autoboxing, ClassUtils.isAssignable(Integer.TYPE, Integer.class));
assertEquals(autoboxing, ClassUtils.isAssignable(Integer.TYPE, Object.class));
assertEquals(autoboxing, ClassUtils.isAssignable(Integer.class, Integer.TYPE));
assertEquals(autoboxing, ClassUtils.isAssignable(Integer.class, Object.class));
assertTrue(ClassUtils.isAssignable(Integer.TYPE, Integer.TYPE));
assertTrue(ClassUtils.isAssignable(Integer.class, Integer.class));
assertEquals(autoboxing, ClassUtils.isAssignable(Boolean.TYPE, Boolean.class));
assertEquals(autoboxing, ClassUtils.isAssignable(Boolean.TYPE, Object.class));
assertEquals(autoboxing, ClassUtils.isAssignable(Boolean.class, Boolean.TYPE));
assertEquals(autoboxing, ClassUtils.isAssignable(Boolean.class, Object.class));
assertTrue(ClassUtils.isAssignable(Boolean.TYPE, Boolean.TYPE));
assertTrue(ClassUtils.isAssignable(Boolean.class, Boolean.class));
}
public void test_isAssignable_Autoboxing() throws Exception {
assertFalse(ClassUtils.isAssignable((Class<?>) null, null, true));
assertFalse(ClassUtils.isAssignable(String.class, null, true));
assertTrue(ClassUtils.isAssignable(null, Object.class, true));
assertTrue(ClassUtils.isAssignable(null, Integer.class, true));
assertFalse(ClassUtils.isAssignable(null, Integer.TYPE, true));
assertTrue(ClassUtils.isAssignable(String.class, Object.class, true));
assertTrue(ClassUtils.isAssignable(String.class, String.class, true));
assertFalse(ClassUtils.isAssignable(Object.class, String.class, true));
assertTrue(ClassUtils.isAssignable(Integer.TYPE, Integer.class, true));
assertTrue(ClassUtils.isAssignable(Integer.TYPE, Object.class, true));
assertTrue(ClassUtils.isAssignable(Integer.class, Integer.TYPE, true));
assertTrue(ClassUtils.isAssignable(Integer.class, Object.class, true));
assertTrue(ClassUtils.isAssignable(Integer.TYPE, Integer.TYPE, true));
assertTrue(ClassUtils.isAssignable(Integer.class, Integer.class, true));
assertTrue(ClassUtils.isAssignable(Boolean.TYPE, Boolean.class, true));
assertTrue(ClassUtils.isAssignable(Boolean.class, Boolean.TYPE, true));
assertTrue(ClassUtils.isAssignable(Boolean.class, Object.class, true));
assertTrue(ClassUtils.isAssignable(Boolean.TYPE, Boolean.TYPE, true));
assertTrue(ClassUtils.isAssignable(Boolean.class, Boolean.class, true));
}
public void test_isAssignable_NoAutoboxing() throws Exception {
assertFalse(ClassUtils.isAssignable((Class<?>) null, null, false));
assertFalse(ClassUtils.isAssignable(String.class, null, false));
assertTrue(ClassUtils.isAssignable(null, Object.class, false));
assertTrue(ClassUtils.isAssignable(null, Integer.class, false));
assertFalse(ClassUtils.isAssignable(null, Integer.TYPE, false));
assertTrue(ClassUtils.isAssignable(String.class, Object.class, false));
assertTrue(ClassUtils.isAssignable(String.class, String.class, false));
assertFalse(ClassUtils.isAssignable(Object.class, String.class, false));
assertFalse(ClassUtils.isAssignable(Integer.TYPE, Integer.class, false));
assertFalse(ClassUtils.isAssignable(Integer.TYPE, Object.class, false));
assertFalse(ClassUtils.isAssignable(Integer.class, Integer.TYPE, false));
assertTrue(ClassUtils.isAssignable(Integer.TYPE, Integer.TYPE, false));
assertTrue(ClassUtils.isAssignable(Integer.class, Integer.class, false));
assertFalse(ClassUtils.isAssignable(Boolean.TYPE, Boolean.class, false));
assertFalse(ClassUtils.isAssignable(Boolean.TYPE, Object.class, false));
assertFalse(ClassUtils.isAssignable(Boolean.class, Boolean.TYPE, false));
assertTrue(ClassUtils.isAssignable(Boolean.class, Object.class, false));
assertTrue(ClassUtils.isAssignable(Boolean.TYPE, Boolean.TYPE, false));
assertTrue(ClassUtils.isAssignable(Boolean.class, Boolean.class, false));
}
public void test_isAssignable_Widening() throws Exception {
// test byte conversions
assertFalse("byte -> char", ClassUtils.isAssignable(Byte.TYPE, Character.TYPE));
assertTrue("byte -> byte", ClassUtils.isAssignable(Byte.TYPE, Byte.TYPE));
assertTrue("byte -> short", ClassUtils.isAssignable(Byte.TYPE, Short.TYPE));
assertTrue("byte -> int", ClassUtils.isAssignable(Byte.TYPE, Integer.TYPE));
assertTrue("byte -> long", ClassUtils.isAssignable(Byte.TYPE, Long.TYPE));
assertTrue("byte -> float", ClassUtils.isAssignable(Byte.TYPE, Float.TYPE));
assertTrue("byte -> double", ClassUtils.isAssignable(Byte.TYPE, Double.TYPE));
assertFalse("byte -> boolean", ClassUtils.isAssignable(Byte.TYPE, Boolean.TYPE));
// test short conversions
assertFalse("short -> char", ClassUtils.isAssignable(Short.TYPE, Character.TYPE));
assertFalse("short -> byte", ClassUtils.isAssignable(Short.TYPE, Byte.TYPE));
assertTrue("short -> short", ClassUtils.isAssignable(Short.TYPE, Short.TYPE));
assertTrue("short -> int", ClassUtils.isAssignable(Short.TYPE, Integer.TYPE));
assertTrue("short -> long", ClassUtils.isAssignable(Short.TYPE, Long.TYPE));
assertTrue("short -> float", ClassUtils.isAssignable(Short.TYPE, Float.TYPE));
assertTrue("short -> double", ClassUtils.isAssignable(Short.TYPE, Double.TYPE));
assertFalse("short -> boolean", ClassUtils.isAssignable(Short.TYPE, Boolean.TYPE));
// test char conversions
assertTrue("char -> char", ClassUtils.isAssignable(Character.TYPE, Character.TYPE));
assertFalse("char -> byte", ClassUtils.isAssignable(Character.TYPE, Byte.TYPE));
assertFalse("char -> short", ClassUtils.isAssignable(Character.TYPE, Short.TYPE));
assertTrue("char -> int", ClassUtils.isAssignable(Character.TYPE, Integer.TYPE));
assertTrue("char -> long", ClassUtils.isAssignable(Character.TYPE, Long.TYPE));
assertTrue("char -> float", ClassUtils.isAssignable(Character.TYPE, Float.TYPE));
assertTrue("char -> double", ClassUtils.isAssignable(Character.TYPE, Double.TYPE));
assertFalse("char -> boolean", ClassUtils.isAssignable(Character.TYPE, Boolean.TYPE));
// test int conversions
assertFalse("int -> char", ClassUtils.isAssignable(Integer.TYPE, Character.TYPE));
assertFalse("int -> byte", ClassUtils.isAssignable(Integer.TYPE, Byte.TYPE));
assertFalse("int -> short", ClassUtils.isAssignable(Integer.TYPE, Short.TYPE));
assertTrue("int -> int", ClassUtils.isAssignable(Integer.TYPE, Integer.TYPE));
assertTrue("int -> long", ClassUtils.isAssignable(Integer.TYPE, Long.TYPE));
assertTrue("int -> float", ClassUtils.isAssignable(Integer.TYPE, Float.TYPE));
assertTrue("int -> double", ClassUtils.isAssignable(Integer.TYPE, Double.TYPE));
assertFalse("int -> boolean", ClassUtils.isAssignable(Integer.TYPE, Boolean.TYPE));
// test long conversions
assertFalse("long -> char", ClassUtils.isAssignable(Long.TYPE, Character.TYPE));
assertFalse("long -> byte", ClassUtils.isAssignable(Long.TYPE, Byte.TYPE));
assertFalse("long -> short", ClassUtils.isAssignable(Long.TYPE, Short.TYPE));
assertFalse("long -> int", ClassUtils.isAssignable(Long.TYPE, Integer.TYPE));
assertTrue("long -> long", ClassUtils.isAssignable(Long.TYPE, Long.TYPE));
assertTrue("long -> float", ClassUtils.isAssignable(Long.TYPE, Float.TYPE));
assertTrue("long -> double", ClassUtils.isAssignable(Long.TYPE, Double.TYPE));
assertFalse("long -> boolean", ClassUtils.isAssignable(Long.TYPE, Boolean.TYPE));
// test float conversions
assertFalse("float -> char", ClassUtils.isAssignable(Float.TYPE, Character.TYPE));
assertFalse("float -> byte", ClassUtils.isAssignable(Float.TYPE, Byte.TYPE));
assertFalse("float -> short", ClassUtils.isAssignable(Float.TYPE, Short.TYPE));
assertFalse("float -> int", ClassUtils.isAssignable(Float.TYPE, Integer.TYPE));
assertFalse("float -> long", ClassUtils.isAssignable(Float.TYPE, Long.TYPE));
assertTrue("float -> float", ClassUtils.isAssignable(Float.TYPE, Float.TYPE));
assertTrue("float -> double", ClassUtils.isAssignable(Float.TYPE, Double.TYPE));
assertFalse("float -> boolean", ClassUtils.isAssignable(Float.TYPE, Boolean.TYPE));
// test double conversions
assertFalse("double -> char", ClassUtils.isAssignable(Double.TYPE, Character.TYPE));
assertFalse("double -> byte", ClassUtils.isAssignable(Double.TYPE, Byte.TYPE));
assertFalse("double -> short", ClassUtils.isAssignable(Double.TYPE, Short.TYPE));
assertFalse("double -> int", ClassUtils.isAssignable(Double.TYPE, Integer.TYPE));
assertFalse("double -> long", ClassUtils.isAssignable(Double.TYPE, Long.TYPE));
assertFalse("double -> float", ClassUtils.isAssignable(Double.TYPE, Float.TYPE));
assertTrue("double -> double", ClassUtils.isAssignable(Double.TYPE, Double.TYPE));
assertFalse("double -> boolean", ClassUtils.isAssignable(Double.TYPE, Boolean.TYPE));
// test boolean conversions
assertFalse("boolean -> char", ClassUtils.isAssignable(Boolean.TYPE, Character.TYPE));
assertFalse("boolean -> byte", ClassUtils.isAssignable(Boolean.TYPE, Byte.TYPE));
assertFalse("boolean -> short", ClassUtils.isAssignable(Boolean.TYPE, Short.TYPE));
assertFalse("boolean -> int", ClassUtils.isAssignable(Boolean.TYPE, Integer.TYPE));
assertFalse("boolean -> long", ClassUtils.isAssignable(Boolean.TYPE, Long.TYPE));
assertFalse("boolean -> float", ClassUtils.isAssignable(Boolean.TYPE, Float.TYPE));
assertFalse("boolean -> double", ClassUtils.isAssignable(Boolean.TYPE, Double.TYPE));
assertTrue("boolean -> boolean", ClassUtils.isAssignable(Boolean.TYPE, Boolean.TYPE));
}
public void test_isAssignable_DefaultUnboxing_Widening() throws Exception {
boolean autoboxing = SystemUtils.isJavaVersionAtLeast(1.5f);
// test byte conversions
assertFalse("byte -> char", ClassUtils.isAssignable(Byte.class, Character.TYPE));
assertEquals("byte -> byte", autoboxing, ClassUtils.isAssignable(Byte.class, Byte.TYPE));
assertEquals("byte -> short", autoboxing, ClassUtils.isAssignable(Byte.class, Short.TYPE));
assertEquals("byte -> int", autoboxing, ClassUtils.isAssignable(Byte.class, Integer.TYPE));
assertEquals("byte -> long", autoboxing, ClassUtils.isAssignable(Byte.class, Long.TYPE));
assertEquals("byte -> float", autoboxing, ClassUtils.isAssignable(Byte.class, Float.TYPE));
assertEquals("byte -> double", autoboxing, ClassUtils.isAssignable(Byte.class, Double.TYPE));
assertFalse("byte -> boolean", ClassUtils.isAssignable(Byte.class, Boolean.TYPE));
// test short conversions
assertFalse("short -> char", ClassUtils.isAssignable(Short.class, Character.TYPE));
assertFalse("short -> byte", ClassUtils.isAssignable(Short.class, Byte.TYPE));
assertEquals("short -> short", autoboxing, ClassUtils.isAssignable(Short.class, Short.TYPE));
assertEquals("short -> int", autoboxing, ClassUtils.isAssignable(Short.class, Integer.TYPE));
assertEquals("short -> long", autoboxing, ClassUtils.isAssignable(Short.class, Long.TYPE));
assertEquals("short -> float", autoboxing, ClassUtils.isAssignable(Short.class, Float.TYPE));
assertEquals("short -> double", autoboxing, ClassUtils.isAssignable(Short.class, Double.TYPE));
assertFalse("short -> boolean", ClassUtils.isAssignable(Short.class, Boolean.TYPE));
// test char conversions
assertEquals("char -> char", autoboxing, ClassUtils.isAssignable(Character.class, Character.TYPE));
assertFalse("char -> byte", ClassUtils.isAssignable(Character.class, Byte.TYPE));
assertFalse("char -> short", ClassUtils.isAssignable(Character.class, Short.TYPE));
assertEquals("char -> int", autoboxing, ClassUtils.isAssignable(Character.class, Integer.TYPE));
assertEquals("char -> long", autoboxing, ClassUtils.isAssignable(Character.class, Long.TYPE));
assertEquals("char -> float", autoboxing, ClassUtils.isAssignable(Character.class, Float.TYPE));
assertEquals("char -> double", autoboxing, ClassUtils.isAssignable(Character.class, Double.TYPE));
assertFalse("char -> boolean", ClassUtils.isAssignable(Character.class, Boolean.TYPE));
// test int conversions
assertFalse("int -> char", ClassUtils.isAssignable(Integer.class, Character.TYPE));
assertFalse("int -> byte", ClassUtils.isAssignable(Integer.class, Byte.TYPE));
assertFalse("int -> short", ClassUtils.isAssignable(Integer.class, Short.TYPE));
assertEquals("int -> int", autoboxing, ClassUtils.isAssignable(Integer.class, Integer.TYPE));
assertEquals("int -> long", autoboxing, ClassUtils.isAssignable(Integer.class, Long.TYPE));
assertEquals("int -> float", autoboxing, ClassUtils.isAssignable(Integer.class, Float.TYPE));
assertEquals("int -> double", autoboxing, ClassUtils.isAssignable(Integer.class, Double.TYPE));
assertFalse("int -> boolean", ClassUtils.isAssignable(Integer.class, Boolean.TYPE));
// test long conversions
assertFalse("long -> char", ClassUtils.isAssignable(Long.class, Character.TYPE));
assertFalse("long -> byte", ClassUtils.isAssignable(Long.class, Byte.TYPE));
assertFalse("long -> short", ClassUtils.isAssignable(Long.class, Short.TYPE));
assertFalse("long -> int", ClassUtils.isAssignable(Long.class, Integer.TYPE));
assertEquals("long -> long", autoboxing, ClassUtils.isAssignable(Long.class, Long.TYPE));
assertEquals("long -> float", autoboxing, ClassUtils.isAssignable(Long.class, Float.TYPE));
assertEquals("long -> double", autoboxing, ClassUtils.isAssignable(Long.class, Double.TYPE));
assertFalse("long -> boolean", ClassUtils.isAssignable(Long.class, Boolean.TYPE));
// test float conversions
assertFalse("float -> char", ClassUtils.isAssignable(Float.class, Character.TYPE));
assertFalse("float -> byte", ClassUtils.isAssignable(Float.class, Byte.TYPE));
assertFalse("float -> short", ClassUtils.isAssignable(Float.class, Short.TYPE));
assertFalse("float -> int", ClassUtils.isAssignable(Float.class, Integer.TYPE));
assertFalse("float -> long", ClassUtils.isAssignable(Float.class, Long.TYPE));
assertEquals("float -> float", autoboxing, ClassUtils.isAssignable(Float.class, Float.TYPE));
assertEquals("float -> double", autoboxing, ClassUtils.isAssignable(Float.class, Double.TYPE));
assertFalse("float -> boolean", ClassUtils.isAssignable(Float.class, Boolean.TYPE));
// test double conversions
assertFalse("double -> char", ClassUtils.isAssignable(Double.class, Character.TYPE));
assertFalse("double -> byte", ClassUtils.isAssignable(Double.class, Byte.TYPE));
assertFalse("double -> short", ClassUtils.isAssignable(Double.class, Short.TYPE));
assertFalse("double -> int", ClassUtils.isAssignable(Double.class, Integer.TYPE));
assertFalse("double -> long", ClassUtils.isAssignable(Double.class, Long.TYPE));
assertFalse("double -> float", ClassUtils.isAssignable(Double.class, Float.TYPE));
assertEquals("double -> double", autoboxing, ClassUtils.isAssignable(Double.class, Double.TYPE));
assertFalse("double -> boolean", ClassUtils.isAssignable(Double.class, Boolean.TYPE));
// test boolean conversions
assertFalse("boolean -> char", ClassUtils.isAssignable(Boolean.class, Character.TYPE));
assertFalse("boolean -> byte", ClassUtils.isAssignable(Boolean.class, Byte.TYPE));
assertFalse("boolean -> short", ClassUtils.isAssignable(Boolean.class, Short.TYPE));
assertFalse("boolean -> int", ClassUtils.isAssignable(Boolean.class, Integer.TYPE));
assertFalse("boolean -> long", ClassUtils.isAssignable(Boolean.class, Long.TYPE));
assertFalse("boolean -> float", ClassUtils.isAssignable(Boolean.class, Float.TYPE));
assertFalse("boolean -> double", ClassUtils.isAssignable(Boolean.class, Double.TYPE));
assertEquals("boolean -> boolean", autoboxing, ClassUtils.isAssignable(Boolean.class, Boolean.TYPE));
}
public void test_isAssignable_Unboxing_Widening() throws Exception {
// test byte conversions
assertFalse("byte -> char", ClassUtils.isAssignable(Byte.class, Character.TYPE, true));
assertTrue("byte -> byte", ClassUtils.isAssignable(Byte.class, Byte.TYPE, true));
assertTrue("byte -> short", ClassUtils.isAssignable(Byte.class, Short.TYPE, true));
assertTrue("byte -> int", ClassUtils.isAssignable(Byte.class, Integer.TYPE, true));
assertTrue("byte -> long", ClassUtils.isAssignable(Byte.class, Long.TYPE, true));
assertTrue("byte -> float", ClassUtils.isAssignable(Byte.class, Float.TYPE, true));
assertTrue("byte -> double", ClassUtils.isAssignable(Byte.class, Double.TYPE, true));
assertFalse("byte -> boolean", ClassUtils.isAssignable(Byte.class, Boolean.TYPE, true));
// test short conversions
assertFalse("short -> char", ClassUtils.isAssignable(Short.class, Character.TYPE, true));
assertFalse("short -> byte", ClassUtils.isAssignable(Short.class, Byte.TYPE, true));
assertTrue("short -> short", ClassUtils.isAssignable(Short.class, Short.TYPE, true));
assertTrue("short -> int", ClassUtils.isAssignable(Short.class, Integer.TYPE, true));
assertTrue("short -> long", ClassUtils.isAssignable(Short.class, Long.TYPE, true));
assertTrue("short -> float", ClassUtils.isAssignable(Short.class, Float.TYPE, true));
assertTrue("short -> double", ClassUtils.isAssignable(Short.class, Double.TYPE, true));
assertFalse("short -> boolean", ClassUtils.isAssignable(Short.class, Boolean.TYPE, true));
// test char conversions
assertTrue("char -> char", ClassUtils.isAssignable(Character.class, Character.TYPE, true));
assertFalse("char -> byte", ClassUtils.isAssignable(Character.class, Byte.TYPE, true));
assertFalse("char -> short", ClassUtils.isAssignable(Character.class, Short.TYPE, true));
assertTrue("char -> int", ClassUtils.isAssignable(Character.class, Integer.TYPE, true));
assertTrue("char -> long", ClassUtils.isAssignable(Character.class, Long.TYPE, true));
assertTrue("char -> float", ClassUtils.isAssignable(Character.class, Float.TYPE, true));
assertTrue("char -> double", ClassUtils.isAssignable(Character.class, Double.TYPE, true));
assertFalse("char -> boolean", ClassUtils.isAssignable(Character.class, Boolean.TYPE, true));
// test int conversions
assertFalse("int -> char", ClassUtils.isAssignable(Integer.class, Character.TYPE, true));
assertFalse("int -> byte", ClassUtils.isAssignable(Integer.class, Byte.TYPE, true));
assertFalse("int -> short", ClassUtils.isAssignable(Integer.class, Short.TYPE, true));
assertTrue("int -> int", ClassUtils.isAssignable(Integer.class, Integer.TYPE, true));
assertTrue("int -> long", ClassUtils.isAssignable(Integer.class, Long.TYPE, true));
assertTrue("int -> float", ClassUtils.isAssignable(Integer.class, Float.TYPE, true));
assertTrue("int -> double", ClassUtils.isAssignable(Integer.class, Double.TYPE, true));
assertFalse("int -> boolean", ClassUtils.isAssignable(Integer.class, Boolean.TYPE, true));
// test long conversions
assertFalse("long -> char", ClassUtils.isAssignable(Long.class, Character.TYPE, true));
assertFalse("long -> byte", ClassUtils.isAssignable(Long.class, Byte.TYPE, true));
assertFalse("long -> short", ClassUtils.isAssignable(Long.class, Short.TYPE, true));
assertFalse("long -> int", ClassUtils.isAssignable(Long.class, Integer.TYPE, true));
assertTrue("long -> long", ClassUtils.isAssignable(Long.class, Long.TYPE, true));
assertTrue("long -> float", ClassUtils.isAssignable(Long.class, Float.TYPE, true));
assertTrue("long -> double", ClassUtils.isAssignable(Long.class, Double.TYPE, true));
assertFalse("long -> boolean", ClassUtils.isAssignable(Long.class, Boolean.TYPE, true));
// test float conversions
assertFalse("float -> char", ClassUtils.isAssignable(Float.class, Character.TYPE, true));
assertFalse("float -> byte", ClassUtils.isAssignable(Float.class, Byte.TYPE, true));
assertFalse("float -> short", ClassUtils.isAssignable(Float.class, Short.TYPE, true));
assertFalse("float -> int", ClassUtils.isAssignable(Float.class, Integer.TYPE, true));
assertFalse("float -> long", ClassUtils.isAssignable(Float.class, Long.TYPE, true));
assertTrue("float -> float", ClassUtils.isAssignable(Float.class, Float.TYPE, true));
assertTrue("float -> double", ClassUtils.isAssignable(Float.class, Double.TYPE, true));
assertFalse("float -> boolean", ClassUtils.isAssignable(Float.class, Boolean.TYPE, true));
// test double conversions
assertFalse("double -> char", ClassUtils.isAssignable(Double.class, Character.TYPE, true));
assertFalse("double -> byte", ClassUtils.isAssignable(Double.class, Byte.TYPE, true));
assertFalse("double -> short", ClassUtils.isAssignable(Double.class, Short.TYPE, true));
assertFalse("double -> int", ClassUtils.isAssignable(Double.class, Integer.TYPE, true));
assertFalse("double -> long", ClassUtils.isAssignable(Double.class, Long.TYPE, true));
assertFalse("double -> float", ClassUtils.isAssignable(Double.class, Float.TYPE, true));
assertTrue("double -> double", ClassUtils.isAssignable(Double.class, Double.TYPE, true));
assertFalse("double -> boolean", ClassUtils.isAssignable(Double.class, Boolean.TYPE, true));
// test boolean conversions
assertFalse("boolean -> char", ClassUtils.isAssignable(Boolean.class, Character.TYPE, true));
assertFalse("boolean -> byte", ClassUtils.isAssignable(Boolean.class, Byte.TYPE, true));
assertFalse("boolean -> short", ClassUtils.isAssignable(Boolean.class, Short.TYPE, true));
assertFalse("boolean -> int", ClassUtils.isAssignable(Boolean.class, Integer.TYPE, true));
assertFalse("boolean -> long", ClassUtils.isAssignable(Boolean.class, Long.TYPE, true));
assertFalse("boolean -> float", ClassUtils.isAssignable(Boolean.class, Float.TYPE, true));
assertFalse("boolean -> double", ClassUtils.isAssignable(Boolean.class, Double.TYPE, true));
assertTrue("boolean -> boolean", ClassUtils.isAssignable(Boolean.class, Boolean.TYPE, true));
}
public void testPrimitiveToWrapper() {
// test primitive classes
assertEquals("boolean -> Boolean.class",
Boolean.class, ClassUtils.primitiveToWrapper(Boolean.TYPE));
assertEquals("byte -> Byte.class",
Byte.class, ClassUtils.primitiveToWrapper(Byte.TYPE));
assertEquals("char -> Character.class",
Character.class, ClassUtils.primitiveToWrapper(Character.TYPE));
assertEquals("short -> Short.class",
Short.class, ClassUtils.primitiveToWrapper(Short.TYPE));
assertEquals("int -> Integer.class",
Integer.class, ClassUtils.primitiveToWrapper(Integer.TYPE));
assertEquals("long -> Long.class",
Long.class, ClassUtils.primitiveToWrapper(Long.TYPE));
assertEquals("double -> Double.class",
Double.class, ClassUtils.primitiveToWrapper(Double.TYPE));
assertEquals("float -> Float.class",
Float.class, ClassUtils.primitiveToWrapper(Float.TYPE));
// test a few other classes
assertEquals("String.class -> String.class",
String.class, ClassUtils.primitiveToWrapper(String.class));
assertEquals("ClassUtils.class -> ClassUtils.class",
org.apache.commons.lang3.ClassUtils.class,
ClassUtils.primitiveToWrapper(org.apache.commons.lang3.ClassUtils.class));
assertEquals("Void.TYPE -> Void.TYPE",
Void.TYPE, ClassUtils.primitiveToWrapper(Void.TYPE));
// test null
assertNull("null -> null",
ClassUtils.primitiveToWrapper(null));
}
public void testPrimitivesToWrappers() {
// test null
assertNull("null -> null",
ClassUtils.primitivesToWrappers(null));
// test empty array
assertEquals("empty -> empty",
ArrayUtils.EMPTY_CLASS_ARRAY, ClassUtils.primitivesToWrappers(ArrayUtils.EMPTY_CLASS_ARRAY));
// test an array of various classes
final Class<?>[] primitives = new Class[] {
Boolean.TYPE, Byte.TYPE, Character.TYPE, Short.TYPE,
Integer.TYPE, Long.TYPE, Double.TYPE, Float.TYPE,
String.class, ClassUtils.class
};
Class<?>[] wrappers= ClassUtils.primitivesToWrappers(primitives);
for (int i=0; i < primitives.length; i++) {
// test each returned wrapper
Class<?> primitive = primitives[i];
Class<?> expectedWrapper = ClassUtils.primitiveToWrapper(primitive);
assertEquals(primitive + " -> " + expectedWrapper, expectedWrapper, wrappers[i]);
}
// test an array of no primitive classes
final Class<?>[] noPrimitives = new Class[] {
String.class, ClassUtils.class, Void.TYPE
};
// This used to return the exact same array, but no longer does.
assertNotSame("unmodified", noPrimitives, ClassUtils.primitivesToWrappers(noPrimitives));
}
public void testWrapperToPrimitive() {
// an array with classes to convert
final Class<?>[] primitives = {
Boolean.TYPE, Byte.TYPE, Character.TYPE, Short.TYPE,
Integer.TYPE, Long.TYPE, Float.TYPE, Double.TYPE
};
for (int i = 0; i < primitives.length; i++) {
Class<?> wrapperCls = ClassUtils.primitiveToWrapper(primitives[i]);
assertFalse("Still primitive", wrapperCls.isPrimitive());
assertEquals(wrapperCls + " -> " + primitives[i], primitives[i],
ClassUtils.wrapperToPrimitive(wrapperCls));
}
}
public void testWrapperToPrimitiveNoWrapper() {
assertNull("Wrong result for non wrapper class", ClassUtils.wrapperToPrimitive(String.class));
}
public void testWrapperToPrimitiveNull() {
assertNull("Wrong result for null class", ClassUtils.wrapperToPrimitive(null));
}
public void testWrappersToPrimitives() {
// an array with classes to test
final Class<?>[] classes = {
Boolean.class, Byte.class, Character.class, Short.class,
Integer.class, Long.class, Float.class, Double.class,
String.class, ClassUtils.class, null
};
Class<?>[] primitives = ClassUtils.wrappersToPrimitives(classes);
// now test the result
assertEquals("Wrong length of result array", classes.length, primitives.length);
for (int i = 0; i < classes.length; i++) {
Class<?> expectedPrimitive = ClassUtils.wrapperToPrimitive(classes[i]);
assertEquals(classes[i] + " -> " + expectedPrimitive, expectedPrimitive,
primitives[i]);
}
}
public void testWrappersToPrimitivesNull() {
assertNull("Wrong result for null input", ClassUtils.wrappersToPrimitives(null));
}
public void testWrappersToPrimitivesEmpty() {
Class<?>[] empty = new Class[0];
assertEquals("Wrong result for empty input", empty, ClassUtils.wrappersToPrimitives(empty));
}
public void testGetClassClassNotFound() throws Exception {
assertGetClassThrowsClassNotFound( "bool" );
assertGetClassThrowsClassNotFound( "bool[]" );
assertGetClassThrowsClassNotFound( "integer[]" );
}
public void testGetClassInvalidArguments() throws Exception {
assertGetClassThrowsNullPointerException( null );
assertGetClassThrowsClassNotFound( "[][][]" );
assertGetClassThrowsClassNotFound( "[[]" );
assertGetClassThrowsClassNotFound( "[" );
assertGetClassThrowsClassNotFound( "java.lang.String][" );
assertGetClassThrowsClassNotFound( ".hello.world" );
assertGetClassThrowsClassNotFound( "hello..world" );
}
public void testWithInterleavingWhitespace() throws ClassNotFoundException {
assertEquals( int[].class, ClassUtils.getClass( " int [ ] " ) );
assertEquals( long[].class, ClassUtils.getClass( "\rlong\t[\n]\r" ) );
assertEquals( short[].class, ClassUtils.getClass( "\tshort \t\t[]" ) );
assertEquals( byte[].class, ClassUtils.getClass( "byte[\t\t\n\r] " ) );
}
public void testGetClassByNormalNameArrays() throws ClassNotFoundException {
assertEquals( int[].class, ClassUtils.getClass( "int[]" ) );
assertEquals( long[].class, ClassUtils.getClass( "long[]" ) );
assertEquals( short[].class, ClassUtils.getClass( "short[]" ) );
assertEquals( byte[].class, ClassUtils.getClass( "byte[]" ) );
assertEquals( char[].class, ClassUtils.getClass( "char[]" ) );
assertEquals( float[].class, ClassUtils.getClass( "float[]" ) );
assertEquals( double[].class, ClassUtils.getClass( "double[]" ) );
assertEquals( boolean[].class, ClassUtils.getClass( "boolean[]" ) );
assertEquals( String[].class, ClassUtils.getClass( "java.lang.String[]" ) );
}
public void testGetClassByNormalNameArrays2D() throws ClassNotFoundException {
assertEquals( int[][].class, ClassUtils.getClass( "int[][]" ) );
assertEquals( long[][].class, ClassUtils.getClass( "long[][]" ) );
assertEquals( short[][].class, ClassUtils.getClass( "short[][]" ) );
assertEquals( byte[][].class, ClassUtils.getClass( "byte[][]" ) );
assertEquals( char[][].class, ClassUtils.getClass( "char[][]" ) );
assertEquals( float[][].class, ClassUtils.getClass( "float[][]" ) );
assertEquals( double[][].class, ClassUtils.getClass( "double[][]" ) );
assertEquals( boolean[][].class, ClassUtils.getClass( "boolean[][]" ) );
assertEquals( String[][].class, ClassUtils.getClass( "java.lang.String[][]" ) );
}
public void testGetClassWithArrayClasses2D() throws Exception {
assertGetClassReturnsClass( String[][].class );
assertGetClassReturnsClass( int[][].class );
assertGetClassReturnsClass( long[][].class );
assertGetClassReturnsClass( short[][].class );
assertGetClassReturnsClass( byte[][].class );
assertGetClassReturnsClass( char[][].class );
assertGetClassReturnsClass( float[][].class );
assertGetClassReturnsClass( double[][].class );
assertGetClassReturnsClass( boolean[][].class );
}
public void testGetClassWithArrayClasses() throws Exception {
assertGetClassReturnsClass( String[].class );
assertGetClassReturnsClass( int[].class );
assertGetClassReturnsClass( long[].class );
assertGetClassReturnsClass( short[].class );
assertGetClassReturnsClass( byte[].class );
assertGetClassReturnsClass( char[].class );
assertGetClassReturnsClass( float[].class );
assertGetClassReturnsClass( double[].class );
assertGetClassReturnsClass( boolean[].class );
}
public void testGetClassRawPrimitives() throws ClassNotFoundException {
assertEquals( int.class, ClassUtils.getClass( "int" ) );
assertEquals( long.class, ClassUtils.getClass( "long" ) );
assertEquals( short.class, ClassUtils.getClass( "short" ) );
assertEquals( byte.class, ClassUtils.getClass( "byte" ) );
assertEquals( char.class, ClassUtils.getClass( "char" ) );
assertEquals( float.class, ClassUtils.getClass( "float" ) );
assertEquals( double.class, ClassUtils.getClass( "double" ) );
assertEquals( boolean.class, ClassUtils.getClass( "boolean" ) );
}
private void assertGetClassReturnsClass( Class<?> c ) throws Exception {
assertEquals( c, ClassUtils.getClass( c.getName() ) );
}
private void assertGetClassThrowsException( String className, Class<?> exceptionType ) throws Exception {
try {
ClassUtils.getClass( className );
fail( "ClassUtils.getClass() should fail with an exception of type " + exceptionType.getName() + " when given class name \"" + className + "\"." );
}
catch( Exception e ) {
assertTrue( exceptionType.isAssignableFrom( e.getClass() ) );
}
}
private void assertGetClassThrowsNullPointerException( String className ) throws Exception {
assertGetClassThrowsException( className, NullPointerException.class );
}
private void assertGetClassThrowsClassNotFound( String className ) throws Exception {
assertGetClassThrowsException( className, ClassNotFoundException.class );
}
// Show the Java bug: http://bugs.sun.com/bugdatabase/view_bug.do?bug_id=4071957
// We may have to delete this if a JDK fixes the bug.
public void testShowJavaBug() throws Exception {
// Tests with Collections$UnmodifiableSet
Set<?> set = Collections.unmodifiableSet(new HashSet<Object>());
Method isEmptyMethod = set.getClass().getMethod("isEmpty", new Class[0]);
try {
isEmptyMethod.invoke(set, new Object[0]);
fail("Failed to throw IllegalAccessException as expected");
} catch(IllegalAccessException iae) {
// expected
}
}
public void testGetPublicMethod() throws Exception {
// Tests with Collections$UnmodifiableSet
Set<?> set = Collections.unmodifiableSet(new HashSet<Object>());
Method isEmptyMethod = ClassUtils.getPublicMethod(set.getClass(), "isEmpty", new Class[0]);
assertTrue(Modifier.isPublic(isEmptyMethod.getDeclaringClass().getModifiers()));
try {
isEmptyMethod.invoke(set, new Object[0]);
} catch(java.lang.IllegalAccessException iae) {
fail("Should not have thrown IllegalAccessException");
}
// Tests with a public Class
Method toStringMethod = ClassUtils.getPublicMethod(Object.class, "toString", new Class[0]);
assertEquals(Object.class.getMethod("toString", new Class[0]), toStringMethod);
}
public void testToClass_object() {
assertNull(ClassUtils.toClass(null));
assertSame(ArrayUtils.EMPTY_CLASS_ARRAY, ClassUtils.toClass(ArrayUtils.EMPTY_OBJECT_ARRAY));
assertTrue(Arrays.equals(new Class[] { String.class, Integer.class, Double.class },
ClassUtils.toClass(new Object[] { "Test", 1, 99d })));
assertTrue(Arrays.equals(new Class[] { String.class, null, Double.class },
ClassUtils.toClass(new Object[] { "Test", null, 99d })));
}
public void test_getShortCanonicalName_Object() {
assertEquals("<null>", ClassUtils.getShortCanonicalName(null, "<null>"));
assertEquals("ClassUtils", ClassUtils.getShortCanonicalName(new ClassUtils(), "<null>"));
assertEquals("ClassUtils[]", ClassUtils.getShortCanonicalName(new ClassUtils[0], "<null>"));
assertEquals("ClassUtils[][]", ClassUtils.getShortCanonicalName(new ClassUtils[0][0], "<null>"));
assertEquals("int[]", ClassUtils.getShortCanonicalName(new int[0], "<null>"));
assertEquals("int[][]", ClassUtils.getShortCanonicalName(new int[0][0], "<null>"));
}
public void test_getShortCanonicalName_Class() {
assertEquals("ClassUtils", ClassUtils.getShortCanonicalName(ClassUtils.class));
assertEquals("ClassUtils[]", ClassUtils.getShortCanonicalName(ClassUtils[].class));
assertEquals("ClassUtils[][]", ClassUtils.getShortCanonicalName(ClassUtils[][].class));
assertEquals("int[]", ClassUtils.getShortCanonicalName(int[].class));
assertEquals("int[][]", ClassUtils.getShortCanonicalName(int[][].class));
}
public void test_getShortCanonicalName_String() {
assertEquals("ClassUtils", ClassUtils.getShortCanonicalName("org.apache.commons.lang3.ClassUtils"));
assertEquals("ClassUtils[]", ClassUtils.getShortCanonicalName("[Lorg.apache.commons.lang3.ClassUtils;"));
assertEquals("ClassUtils[][]", ClassUtils.getShortCanonicalName("[[Lorg.apache.commons.lang3.ClassUtils;"));
assertEquals("ClassUtils[]", ClassUtils.getShortCanonicalName("org.apache.commons.lang3.ClassUtils[]"));
assertEquals("ClassUtils[][]", ClassUtils.getShortCanonicalName("org.apache.commons.lang3.ClassUtils[][]"));
assertEquals("int[]", ClassUtils.getShortCanonicalName("[I"));
assertEquals("int[][]", ClassUtils.getShortCanonicalName("[[I"));
assertEquals("int[]", ClassUtils.getShortCanonicalName("int[]"));
assertEquals("int[][]", ClassUtils.getShortCanonicalName("int[][]"));
}
public void test_getPackageCanonicalName_Object() {
assertEquals("<null>", ClassUtils.getPackageCanonicalName(null, "<null>"));
assertEquals("org.apache.commons.lang3", ClassUtils.getPackageCanonicalName(new ClassUtils(), "<null>"));
assertEquals("org.apache.commons.lang3", ClassUtils.getPackageCanonicalName(new ClassUtils[0], "<null>"));
assertEquals("org.apache.commons.lang3", ClassUtils.getPackageCanonicalName(new ClassUtils[0][0], "<null>"));
assertEquals("", ClassUtils.getPackageCanonicalName(new int[0], "<null>"));
assertEquals("", ClassUtils.getPackageCanonicalName(new int[0][0], "<null>"));
}
public void test_getPackageCanonicalName_Class() {
assertEquals("org.apache.commons.lang3", ClassUtils.getPackageCanonicalName(ClassUtils.class));
assertEquals("org.apache.commons.lang3", ClassUtils.getPackageCanonicalName(ClassUtils[].class));
assertEquals("org.apache.commons.lang3", ClassUtils.getPackageCanonicalName(ClassUtils[][].class));
assertEquals("", ClassUtils.getPackageCanonicalName(int[].class));
assertEquals("", ClassUtils.getPackageCanonicalName(int[][].class));
}
public void test_getPackageCanonicalName_String() {
assertEquals("org.apache.commons.lang3",
ClassUtils.getPackageCanonicalName("org.apache.commons.lang3.ClassUtils"));
assertEquals("org.apache.commons.lang3",
ClassUtils.getPackageCanonicalName("[Lorg.apache.commons.lang3.ClassUtils;"));
assertEquals("org.apache.commons.lang3",
ClassUtils.getPackageCanonicalName("[[Lorg.apache.commons.lang3.ClassUtils;"));
assertEquals("org.apache.commons.lang3",
ClassUtils.getPackageCanonicalName("org.apache.commons.lang3.ClassUtils[]"));
assertEquals("org.apache.commons.lang3",
ClassUtils.getPackageCanonicalName("org.apache.commons.lang3.ClassUtils[][]"));
assertEquals("", ClassUtils.getPackageCanonicalName("[I"));
assertEquals("", ClassUtils.getPackageCanonicalName("[[I"));
assertEquals("", ClassUtils.getPackageCanonicalName("int[]"));
assertEquals("", ClassUtils.getPackageCanonicalName("int[][]"));
}
} |
||
@Test
public void testCreateNumber() {
// a lot of things can go wrong
assertEquals("createNumber(String) 1 failed", Float.valueOf("1234.5"), NumberUtils.createNumber("1234.5"));
assertEquals("createNumber(String) 2 failed", Integer.valueOf("12345"), NumberUtils.createNumber("12345"));
assertEquals("createNumber(String) 3 failed", Double.valueOf("1234.5"), NumberUtils.createNumber("1234.5D"));
assertEquals("createNumber(String) 3 failed", Double.valueOf("1234.5"), NumberUtils.createNumber("1234.5d"));
assertEquals("createNumber(String) 4 failed", Float.valueOf("1234.5"), NumberUtils.createNumber("1234.5F"));
assertEquals("createNumber(String) 4 failed", Float.valueOf("1234.5"), NumberUtils.createNumber("1234.5f"));
assertEquals("createNumber(String) 5 failed", Long.valueOf(Integer.MAX_VALUE + 1L), NumberUtils.createNumber(""
+ (Integer.MAX_VALUE + 1L)));
assertEquals("createNumber(String) 6 failed", Long.valueOf(12345), NumberUtils.createNumber("12345L"));
assertEquals("createNumber(String) 6 failed", Long.valueOf(12345), NumberUtils.createNumber("12345l"));
assertEquals("createNumber(String) 7 failed", Float.valueOf("-1234.5"), NumberUtils.createNumber("-1234.5"));
assertEquals("createNumber(String) 8 failed", Integer.valueOf("-12345"), NumberUtils.createNumber("-12345"));
assertTrue("createNumber(String) 9a failed", 0xFADE == NumberUtils.createNumber("0xFADE").intValue());
assertTrue("createNumber(String) 9b failed", 0xFADE == NumberUtils.createNumber("0Xfade").intValue());
assertTrue("createNumber(String) 10a failed", -0xFADE == NumberUtils.createNumber("-0xFADE").intValue());
assertTrue("createNumber(String) 10b failed", -0xFADE == NumberUtils.createNumber("-0Xfade").intValue());
assertEquals("createNumber(String) 11 failed", Double.valueOf("1.1E200"), NumberUtils.createNumber("1.1E200"));
assertEquals("createNumber(String) 12 failed", Float.valueOf("1.1E20"), NumberUtils.createNumber("1.1E20"));
assertEquals("createNumber(String) 13 failed", Double.valueOf("-1.1E200"), NumberUtils.createNumber("-1.1E200"));
assertEquals("createNumber(String) 14 failed", Double.valueOf("1.1E-200"), NumberUtils.createNumber("1.1E-200"));
assertEquals("createNumber(null) failed", null, NumberUtils.createNumber(null));
assertEquals("createNumber(String) failed", new BigInteger("12345678901234567890"), NumberUtils
.createNumber("12345678901234567890L"));
// jdk 1.2 doesn't support this. unsure about jdk 1.2.2
if (SystemUtils.isJavaVersionAtLeast(JAVA_1_3)) {
assertEquals("createNumber(String) 15 failed", new BigDecimal("1.1E-700"), NumberUtils
.createNumber("1.1E-700F"));
}
assertEquals("createNumber(String) 16 failed", Long.valueOf("10" + Integer.MAX_VALUE), NumberUtils
.createNumber("10" + Integer.MAX_VALUE + "L"));
assertEquals("createNumber(String) 17 failed", Long.valueOf("10" + Integer.MAX_VALUE), NumberUtils
.createNumber("10" + Integer.MAX_VALUE));
assertEquals("createNumber(String) 18 failed", new BigInteger("10" + Long.MAX_VALUE), NumberUtils
.createNumber("10" + Long.MAX_VALUE));
// LANG-521
assertEquals("createNumber(String) LANG-521 failed", Float.valueOf("2."), NumberUtils.createNumber("2."));
// LANG-638
assertFalse("createNumber(String) succeeded", checkCreateNumber("1eE"));
// LANG-693
assertEquals("createNumber(String) LANG-693 failed", Double.valueOf(Double.MAX_VALUE), NumberUtils
.createNumber("" + Double.MAX_VALUE));
} | org.apache.commons.lang3.math.NumberUtilsTest::testCreateNumber | src/test/java/org/apache/commons/lang3/math/NumberUtilsTest.java | 228 | src/test/java/org/apache/commons/lang3/math/NumberUtilsTest.java | testCreateNumber | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.lang3.math;
import static org.apache.commons.lang3.JavaVersion.JAVA_1_3;
import static org.junit.Assert.*;
import java.lang.reflect.Constructor;
import java.lang.reflect.Modifier;
import java.math.BigDecimal;
import java.math.BigInteger;
import org.apache.commons.lang3.SystemUtils;
import org.junit.Test;
/**
* Unit tests {@link org.apache.commons.lang3.math.NumberUtils}.
*
* @version $Id$
*/
public class NumberUtilsTest {
//-----------------------------------------------------------------------
@Test
public void testConstructor() {
assertNotNull(new NumberUtils());
Constructor<?>[] cons = NumberUtils.class.getDeclaredConstructors();
assertEquals(1, cons.length);
assertTrue(Modifier.isPublic(cons[0].getModifiers()));
assertTrue(Modifier.isPublic(NumberUtils.class.getModifiers()));
assertFalse(Modifier.isFinal(NumberUtils.class.getModifiers()));
}
//---------------------------------------------------------------------
/**
* Test for {@link NumberUtils#toInt(String)}.
*/
@Test
public void testToIntString() {
assertTrue("toInt(String) 1 failed", NumberUtils.toInt("12345") == 12345);
assertTrue("toInt(String) 2 failed", NumberUtils.toInt("abc") == 0);
assertTrue("toInt(empty) failed", NumberUtils.toInt("") == 0);
assertTrue("toInt(null) failed", NumberUtils.toInt(null) == 0);
}
/**
* Test for {@link NumberUtils#toInt(String, int)}.
*/
@Test
public void testToIntStringI() {
assertTrue("toInt(String,int) 1 failed", NumberUtils.toInt("12345", 5) == 12345);
assertTrue("toInt(String,int) 2 failed", NumberUtils.toInt("1234.5", 5) == 5);
}
/**
* Test for {@link NumberUtils#toLong(String)}.
*/
@Test
public void testToLongString() {
assertTrue("toLong(String) 1 failed", NumberUtils.toLong("12345") == 12345l);
assertTrue("toLong(String) 2 failed", NumberUtils.toLong("abc") == 0l);
assertTrue("toLong(String) 3 failed", NumberUtils.toLong("1L") == 0l);
assertTrue("toLong(String) 4 failed", NumberUtils.toLong("1l") == 0l);
assertTrue("toLong(Long.MAX_VALUE) failed", NumberUtils.toLong(Long.MAX_VALUE+"") == Long.MAX_VALUE);
assertTrue("toLong(Long.MIN_VALUE) failed", NumberUtils.toLong(Long.MIN_VALUE+"") == Long.MIN_VALUE);
assertTrue("toLong(empty) failed", NumberUtils.toLong("") == 0l);
assertTrue("toLong(null) failed", NumberUtils.toLong(null) == 0l);
}
/**
* Test for {@link NumberUtils#toLong(String, long)}.
*/
@Test
public void testToLongStringL() {
assertTrue("toLong(String,long) 1 failed", NumberUtils.toLong("12345", 5l) == 12345l);
assertTrue("toLong(String,long) 2 failed", NumberUtils.toLong("1234.5", 5l) == 5l);
}
/**
* Test for {@link NumberUtils#toFloat(String)}.
*/
@Test
public void testToFloatString() {
assertTrue("toFloat(String) 1 failed", NumberUtils.toFloat("-1.2345") == -1.2345f);
assertTrue("toFloat(String) 2 failed", NumberUtils.toFloat("1.2345") == 1.2345f);
assertTrue("toFloat(String) 3 failed", NumberUtils.toFloat("abc") == 0.0f);
assertTrue("toFloat(Float.MAX_VALUE) failed", NumberUtils.toFloat(Float.MAX_VALUE+"") == Float.MAX_VALUE);
assertTrue("toFloat(Float.MIN_VALUE) failed", NumberUtils.toFloat(Float.MIN_VALUE+"") == Float.MIN_VALUE);
assertTrue("toFloat(empty) failed", NumberUtils.toFloat("") == 0.0f);
assertTrue("toFloat(null) failed", NumberUtils.toFloat(null) == 0.0f);
}
/**
* Test for {@link NumberUtils#toFloat(String, float)}.
*/
@Test
public void testToFloatStringF() {
assertTrue("toFloat(String,int) 1 failed", NumberUtils.toFloat("1.2345", 5.1f) == 1.2345f);
assertTrue("toFloat(String,int) 2 failed", NumberUtils.toFloat("a", 5.0f) == 5.0f);
}
/**
* Test for {@link NumberUtils#toDouble(String)}.
*/
@Test
public void testStringToDoubleString() {
assertTrue("toDouble(String) 1 failed", NumberUtils.toDouble("-1.2345") == -1.2345d);
assertTrue("toDouble(String) 2 failed", NumberUtils.toDouble("1.2345") == 1.2345d);
assertTrue("toDouble(String) 3 failed", NumberUtils.toDouble("abc") == 0.0d);
assertTrue("toDouble(Double.MAX_VALUE) failed", NumberUtils.toDouble(Double.MAX_VALUE+"") == Double.MAX_VALUE);
assertTrue("toDouble(Double.MIN_VALUE) failed", NumberUtils.toDouble(Double.MIN_VALUE+"") == Double.MIN_VALUE);
assertTrue("toDouble(empty) failed", NumberUtils.toDouble("") == 0.0d);
assertTrue("toDouble(null) failed", NumberUtils.toDouble(null) == 0.0d);
}
/**
* Test for {@link NumberUtils#toDouble(String, double)}.
*/
@Test
public void testStringToDoubleStringD() {
assertTrue("toDouble(String,int) 1 failed", NumberUtils.toDouble("1.2345", 5.1d) == 1.2345d);
assertTrue("toDouble(String,int) 2 failed", NumberUtils.toDouble("a", 5.0d) == 5.0d);
}
/**
* Test for {@link NumberUtils#toByte(String)}.
*/
@Test
public void testToByteString() {
assertTrue("toByte(String) 1 failed", NumberUtils.toByte("123") == 123);
assertTrue("toByte(String) 2 failed", NumberUtils.toByte("abc") == 0);
assertTrue("toByte(empty) failed", NumberUtils.toByte("") == 0);
assertTrue("toByte(null) failed", NumberUtils.toByte(null) == 0);
}
/**
* Test for {@link NumberUtils#toByte(String, byte)}.
*/
@Test
public void testToByteStringI() {
assertTrue("toByte(String,byte) 1 failed", NumberUtils.toByte("123", (byte) 5) == 123);
assertTrue("toByte(String,byte) 2 failed", NumberUtils.toByte("12.3", (byte) 5) == 5);
}
/**
* Test for {@link NumberUtils#toShort(String)}.
*/
@Test
public void testToShortString() {
assertTrue("toShort(String) 1 failed", NumberUtils.toShort("12345") == 12345);
assertTrue("toShort(String) 2 failed", NumberUtils.toShort("abc") == 0);
assertTrue("toShort(empty) failed", NumberUtils.toShort("") == 0);
assertTrue("toShort(null) failed", NumberUtils.toShort(null) == 0);
}
/**
* Test for {@link NumberUtils#toShort(String, short)}.
*/
@Test
public void testToShortStringI() {
assertTrue("toShort(String,short) 1 failed", NumberUtils.toShort("12345", (short) 5) == 12345);
assertTrue("toShort(String,short) 2 failed", NumberUtils.toShort("1234.5", (short) 5) == 5);
}
@Test
public void testCreateNumber() {
// a lot of things can go wrong
assertEquals("createNumber(String) 1 failed", Float.valueOf("1234.5"), NumberUtils.createNumber("1234.5"));
assertEquals("createNumber(String) 2 failed", Integer.valueOf("12345"), NumberUtils.createNumber("12345"));
assertEquals("createNumber(String) 3 failed", Double.valueOf("1234.5"), NumberUtils.createNumber("1234.5D"));
assertEquals("createNumber(String) 3 failed", Double.valueOf("1234.5"), NumberUtils.createNumber("1234.5d"));
assertEquals("createNumber(String) 4 failed", Float.valueOf("1234.5"), NumberUtils.createNumber("1234.5F"));
assertEquals("createNumber(String) 4 failed", Float.valueOf("1234.5"), NumberUtils.createNumber("1234.5f"));
assertEquals("createNumber(String) 5 failed", Long.valueOf(Integer.MAX_VALUE + 1L), NumberUtils.createNumber(""
+ (Integer.MAX_VALUE + 1L)));
assertEquals("createNumber(String) 6 failed", Long.valueOf(12345), NumberUtils.createNumber("12345L"));
assertEquals("createNumber(String) 6 failed", Long.valueOf(12345), NumberUtils.createNumber("12345l"));
assertEquals("createNumber(String) 7 failed", Float.valueOf("-1234.5"), NumberUtils.createNumber("-1234.5"));
assertEquals("createNumber(String) 8 failed", Integer.valueOf("-12345"), NumberUtils.createNumber("-12345"));
assertTrue("createNumber(String) 9a failed", 0xFADE == NumberUtils.createNumber("0xFADE").intValue());
assertTrue("createNumber(String) 9b failed", 0xFADE == NumberUtils.createNumber("0Xfade").intValue());
assertTrue("createNumber(String) 10a failed", -0xFADE == NumberUtils.createNumber("-0xFADE").intValue());
assertTrue("createNumber(String) 10b failed", -0xFADE == NumberUtils.createNumber("-0Xfade").intValue());
assertEquals("createNumber(String) 11 failed", Double.valueOf("1.1E200"), NumberUtils.createNumber("1.1E200"));
assertEquals("createNumber(String) 12 failed", Float.valueOf("1.1E20"), NumberUtils.createNumber("1.1E20"));
assertEquals("createNumber(String) 13 failed", Double.valueOf("-1.1E200"), NumberUtils.createNumber("-1.1E200"));
assertEquals("createNumber(String) 14 failed", Double.valueOf("1.1E-200"), NumberUtils.createNumber("1.1E-200"));
assertEquals("createNumber(null) failed", null, NumberUtils.createNumber(null));
assertEquals("createNumber(String) failed", new BigInteger("12345678901234567890"), NumberUtils
.createNumber("12345678901234567890L"));
// jdk 1.2 doesn't support this. unsure about jdk 1.2.2
if (SystemUtils.isJavaVersionAtLeast(JAVA_1_3)) {
assertEquals("createNumber(String) 15 failed", new BigDecimal("1.1E-700"), NumberUtils
.createNumber("1.1E-700F"));
}
assertEquals("createNumber(String) 16 failed", Long.valueOf("10" + Integer.MAX_VALUE), NumberUtils
.createNumber("10" + Integer.MAX_VALUE + "L"));
assertEquals("createNumber(String) 17 failed", Long.valueOf("10" + Integer.MAX_VALUE), NumberUtils
.createNumber("10" + Integer.MAX_VALUE));
assertEquals("createNumber(String) 18 failed", new BigInteger("10" + Long.MAX_VALUE), NumberUtils
.createNumber("10" + Long.MAX_VALUE));
// LANG-521
assertEquals("createNumber(String) LANG-521 failed", Float.valueOf("2."), NumberUtils.createNumber("2."));
// LANG-638
assertFalse("createNumber(String) succeeded", checkCreateNumber("1eE"));
// LANG-693
assertEquals("createNumber(String) LANG-693 failed", Double.valueOf(Double.MAX_VALUE), NumberUtils
.createNumber("" + Double.MAX_VALUE));
}
@Test
public void testCreateFloat() {
assertEquals("createFloat(String) failed", Float.valueOf("1234.5"), NumberUtils.createFloat("1234.5"));
assertEquals("createFloat(null) failed", null, NumberUtils.createFloat(null));
this.testCreateFloatFailure("");
this.testCreateFloatFailure(" ");
this.testCreateFloatFailure("\b\t\n\f\r");
// Funky whitespaces
this.testCreateFloatFailure("\u00A0\uFEFF\u000B\u000C\u001C\u001D\u001E\u001F");
}
protected void testCreateFloatFailure(String str) {
try {
Float value = NumberUtils.createFloat(str);
fail("createFloat(blank) failed: " + value);
} catch (NumberFormatException ex) {
// empty
}
}
@Test
public void testCreateDouble() {
assertEquals("createDouble(String) failed", Double.valueOf("1234.5"), NumberUtils.createDouble("1234.5"));
assertEquals("createDouble(null) failed", null, NumberUtils.createDouble(null));
this.testCreateDoubleFailure("");
this.testCreateDoubleFailure(" ");
this.testCreateDoubleFailure("\b\t\n\f\r");
// Funky whitespaces
this.testCreateDoubleFailure("\u00A0\uFEFF\u000B\u000C\u001C\u001D\u001E\u001F");
}
protected void testCreateDoubleFailure(String str) {
try {
Double value = NumberUtils.createDouble(str);
fail("createDouble(blank) failed: " + value);
} catch (NumberFormatException ex) {
// empty
}
}
@Test
public void testCreateInteger() {
assertEquals("createInteger(String) failed", Integer.valueOf("12345"), NumberUtils.createInteger("12345"));
assertEquals("createInteger(null) failed", null, NumberUtils.createInteger(null));
this.testCreateIntegerFailure("");
this.testCreateIntegerFailure(" ");
this.testCreateIntegerFailure("\b\t\n\f\r");
// Funky whitespaces
this.testCreateIntegerFailure("\u00A0\uFEFF\u000B\u000C\u001C\u001D\u001E\u001F");
}
protected void testCreateIntegerFailure(String str) {
try {
Integer value = NumberUtils.createInteger(str);
fail("createInteger(blank) failed: " + value);
} catch (NumberFormatException ex) {
// empty
}
}
@Test
public void testCreateLong() {
assertEquals("createLong(String) failed", Long.valueOf("12345"), NumberUtils.createLong("12345"));
assertEquals("createLong(null) failed", null, NumberUtils.createLong(null));
this.testCreateLongFailure("");
this.testCreateLongFailure(" ");
this.testCreateLongFailure("\b\t\n\f\r");
// Funky whitespaces
this.testCreateLongFailure("\u00A0\uFEFF\u000B\u000C\u001C\u001D\u001E\u001F");
}
protected void testCreateLongFailure(String str) {
try {
Long value = NumberUtils.createLong(str);
fail("createLong(blank) failed: " + value);
} catch (NumberFormatException ex) {
// empty
}
}
@Test
public void testCreateBigInteger() {
assertEquals("createBigInteger(String) failed", new BigInteger("12345"), NumberUtils.createBigInteger("12345"));
assertEquals("createBigInteger(null) failed", null, NumberUtils.createBigInteger(null));
this.testCreateBigIntegerFailure("");
this.testCreateBigIntegerFailure(" ");
this.testCreateBigIntegerFailure("\b\t\n\f\r");
// Funky whitespaces
this.testCreateBigIntegerFailure("\u00A0\uFEFF\u000B\u000C\u001C\u001D\u001E\u001F");
}
protected void testCreateBigIntegerFailure(String str) {
try {
BigInteger value = NumberUtils.createBigInteger(str);
fail("createBigInteger(blank) failed: " + value);
} catch (NumberFormatException ex) {
// empty
}
}
@Test
public void testCreateBigDecimal() {
assertEquals("createBigDecimal(String) failed", new BigDecimal("1234.5"), NumberUtils.createBigDecimal("1234.5"));
assertEquals("createBigDecimal(null) failed", null, NumberUtils.createBigDecimal(null));
this.testCreateBigDecimalFailure("");
this.testCreateBigDecimalFailure(" ");
this.testCreateBigDecimalFailure("\b\t\n\f\r");
// Funky whitespaces
this.testCreateBigDecimalFailure("\u00A0\uFEFF\u000B\u000C\u001C\u001D\u001E\u001F");
}
protected void testCreateBigDecimalFailure(String str) {
try {
BigDecimal value = NumberUtils.createBigDecimal(str);
fail("createBigDecimal(blank) failed: " + value);
} catch (NumberFormatException ex) {
// empty
}
}
// min/max tests
// ----------------------------------------------------------------------
@Test(expected = IllegalArgumentException.class)
public void testMinLong_nullArray() {
NumberUtils.min((long[]) null);
}
@Test(expected = IllegalArgumentException.class)
public void testMinLong_emptyArray() {
NumberUtils.min(new long[0]);
}
@Test
public void testMinLong() {
assertEquals(
"min(long[]) failed for array length 1",
5,
NumberUtils.min(new long[] { 5 }));
assertEquals(
"min(long[]) failed for array length 2",
6,
NumberUtils.min(new long[] { 6, 9 }));
assertEquals(-10, NumberUtils.min(new long[] { -10, -5, 0, 5, 10 }));
assertEquals(-10, NumberUtils.min(new long[] { -5, 0, -10, 5, 10 }));
}
@Test(expected = IllegalArgumentException.class)
public void testMinInt_nullArray() {
NumberUtils.min((int[]) null);
}
@Test(expected = IllegalArgumentException.class)
public void testMinInt_emptyArray() {
NumberUtils.min(new int[0]);
}
@Test
public void testMinInt() {
assertEquals(
"min(int[]) failed for array length 1",
5,
NumberUtils.min(new int[] { 5 }));
assertEquals(
"min(int[]) failed for array length 2",
6,
NumberUtils.min(new int[] { 6, 9 }));
assertEquals(-10, NumberUtils.min(new int[] { -10, -5, 0, 5, 10 }));
assertEquals(-10, NumberUtils.min(new int[] { -5, 0, -10, 5, 10 }));
}
@Test(expected = IllegalArgumentException.class)
public void testMinShort_nullArray() {
NumberUtils.min((short[]) null);
}
@Test(expected = IllegalArgumentException.class)
public void testMinShort_emptyArray() {
NumberUtils.min(new short[0]);
}
@Test
public void testMinShort() {
assertEquals(
"min(short[]) failed for array length 1",
5,
NumberUtils.min(new short[] { 5 }));
assertEquals(
"min(short[]) failed for array length 2",
6,
NumberUtils.min(new short[] { 6, 9 }));
assertEquals(-10, NumberUtils.min(new short[] { -10, -5, 0, 5, 10 }));
assertEquals(-10, NumberUtils.min(new short[] { -5, 0, -10, 5, 10 }));
}
@Test(expected = IllegalArgumentException.class)
public void testMinByte_nullArray() {
NumberUtils.min((byte[]) null);
}
@Test(expected = IllegalArgumentException.class)
public void testMinByte_emptyArray() {
NumberUtils.min(new byte[0]);
}
@Test
public void testMinByte() {
assertEquals(
"min(byte[]) failed for array length 1",
5,
NumberUtils.min(new byte[] { 5 }));
assertEquals(
"min(byte[]) failed for array length 2",
6,
NumberUtils.min(new byte[] { 6, 9 }));
assertEquals(-10, NumberUtils.min(new byte[] { -10, -5, 0, 5, 10 }));
assertEquals(-10, NumberUtils.min(new byte[] { -5, 0, -10, 5, 10 }));
}
@Test(expected = IllegalArgumentException.class)
public void testMinDouble_nullArray() {
NumberUtils.min((double[]) null);
}
@Test(expected = IllegalArgumentException.class)
public void testMinDouble_emptyArray() {
NumberUtils.min(new double[0]);
}
@Test
public void testMinDouble() {
assertEquals(
"min(double[]) failed for array length 1",
5.12,
NumberUtils.min(new double[] { 5.12 }),
0);
assertEquals(
"min(double[]) failed for array length 2",
6.23,
NumberUtils.min(new double[] { 6.23, 9.34 }),
0);
assertEquals(
"min(double[]) failed for array length 5",
-10.45,
NumberUtils.min(new double[] { -10.45, -5.56, 0, 5.67, 10.78 }),
0);
assertEquals(-10, NumberUtils.min(new double[] { -10, -5, 0, 5, 10 }), 0.0001);
assertEquals(-10, NumberUtils.min(new double[] { -5, 0, -10, 5, 10 }), 0.0001);
}
@Test(expected = IllegalArgumentException.class)
public void testMinFloat_nullArray() {
NumberUtils.min((float[]) null);
}
@Test(expected = IllegalArgumentException.class)
public void testMinFloat_emptyArray() {
NumberUtils.min(new float[0]);
}
@Test
public void testMinFloat() {
assertEquals(
"min(float[]) failed for array length 1",
5.9f,
NumberUtils.min(new float[] { 5.9f }),
0);
assertEquals(
"min(float[]) failed for array length 2",
6.8f,
NumberUtils.min(new float[] { 6.8f, 9.7f }),
0);
assertEquals(
"min(float[]) failed for array length 5",
-10.6f,
NumberUtils.min(new float[] { -10.6f, -5.5f, 0, 5.4f, 10.3f }),
0);
assertEquals(-10, NumberUtils.min(new float[] { -10, -5, 0, 5, 10 }), 0.0001f);
assertEquals(-10, NumberUtils.min(new float[] { -5, 0, -10, 5, 10 }), 0.0001f);
}
@Test(expected = IllegalArgumentException.class)
public void testMaxLong_nullArray() {
NumberUtils.max((long[]) null);
}
@Test(expected = IllegalArgumentException.class)
public void testMaxLong_emptyArray() {
NumberUtils.max(new long[0]);
}
@Test
public void testMaxLong() {
assertEquals(
"max(long[]) failed for array length 1",
5,
NumberUtils.max(new long[] { 5 }));
assertEquals(
"max(long[]) failed for array length 2",
9,
NumberUtils.max(new long[] { 6, 9 }));
assertEquals(
"max(long[]) failed for array length 5",
10,
NumberUtils.max(new long[] { -10, -5, 0, 5, 10 }));
assertEquals(10, NumberUtils.max(new long[] { -10, -5, 0, 5, 10 }));
assertEquals(10, NumberUtils.max(new long[] { -5, 0, 10, 5, -10 }));
}
@Test(expected = IllegalArgumentException.class)
public void testMaxInt_nullArray() {
NumberUtils.max((int[]) null);
}
@Test(expected = IllegalArgumentException.class)
public void testMaxInt_emptyArray() {
NumberUtils.max(new int[0]);
}
@Test
public void testMaxInt() {
assertEquals(
"max(int[]) failed for array length 1",
5,
NumberUtils.max(new int[] { 5 }));
assertEquals(
"max(int[]) failed for array length 2",
9,
NumberUtils.max(new int[] { 6, 9 }));
assertEquals(
"max(int[]) failed for array length 5",
10,
NumberUtils.max(new int[] { -10, -5, 0, 5, 10 }));
assertEquals(10, NumberUtils.max(new int[] { -10, -5, 0, 5, 10 }));
assertEquals(10, NumberUtils.max(new int[] { -5, 0, 10, 5, -10 }));
}
@Test(expected = IllegalArgumentException.class)
public void testMaxShort_nullArray() {
NumberUtils.max((short[]) null);
}
@Test(expected = IllegalArgumentException.class)
public void testMaxShort_emptyArray() {
NumberUtils.max(new short[0]);
}
@Test
public void testMaxShort() {
assertEquals(
"max(short[]) failed for array length 1",
5,
NumberUtils.max(new short[] { 5 }));
assertEquals(
"max(short[]) failed for array length 2",
9,
NumberUtils.max(new short[] { 6, 9 }));
assertEquals(
"max(short[]) failed for array length 5",
10,
NumberUtils.max(new short[] { -10, -5, 0, 5, 10 }));
assertEquals(10, NumberUtils.max(new short[] { -10, -5, 0, 5, 10 }));
assertEquals(10, NumberUtils.max(new short[] { -5, 0, 10, 5, -10 }));
}
@Test(expected = IllegalArgumentException.class)
public void testMaxByte_nullArray() {
NumberUtils.max((byte[]) null);
}
@Test(expected = IllegalArgumentException.class)
public void testMaxByte_emptyArray() {
NumberUtils.max(new byte[0]);
}
@Test
public void testMaxByte() {
assertEquals(
"max(byte[]) failed for array length 1",
5,
NumberUtils.max(new byte[] { 5 }));
assertEquals(
"max(byte[]) failed for array length 2",
9,
NumberUtils.max(new byte[] { 6, 9 }));
assertEquals(
"max(byte[]) failed for array length 5",
10,
NumberUtils.max(new byte[] { -10, -5, 0, 5, 10 }));
assertEquals(10, NumberUtils.max(new byte[] { -10, -5, 0, 5, 10 }));
assertEquals(10, NumberUtils.max(new byte[] { -5, 0, 10, 5, -10 }));
}
@Test(expected = IllegalArgumentException.class)
public void testMaxDouble_nullArray() {
NumberUtils.max((double[]) null);
}
@Test(expected = IllegalArgumentException.class)
public void testMaxDouble_emptyArray() {
NumberUtils.max(new double[0]);
}
@Test
public void testMaxDouble() {
final double[] d = null;
try {
NumberUtils.max(d);
fail("No exception was thrown for null input.");
} catch (IllegalArgumentException ex) {}
try {
NumberUtils.max(new double[0]);
fail("No exception was thrown for empty input.");
} catch (IllegalArgumentException ex) {}
assertEquals(
"max(double[]) failed for array length 1",
5.1f,
NumberUtils.max(new double[] { 5.1f }),
0);
assertEquals(
"max(double[]) failed for array length 2",
9.2f,
NumberUtils.max(new double[] { 6.3f, 9.2f }),
0);
assertEquals(
"max(double[]) failed for float length 5",
10.4f,
NumberUtils.max(new double[] { -10.5f, -5.6f, 0, 5.7f, 10.4f }),
0);
assertEquals(10, NumberUtils.max(new double[] { -10, -5, 0, 5, 10 }), 0.0001);
assertEquals(10, NumberUtils.max(new double[] { -5, 0, 10, 5, -10 }), 0.0001);
}
@Test(expected = IllegalArgumentException.class)
public void testMaxFloat_nullArray() {
NumberUtils.max((float[]) null);
}
@Test(expected = IllegalArgumentException.class)
public void testMaxFloat_emptyArray() {
NumberUtils.max(new float[0]);
}
@Test
public void testMaxFloat() {
assertEquals(
"max(float[]) failed for array length 1",
5.1f,
NumberUtils.max(new float[] { 5.1f }),
0);
assertEquals(
"max(float[]) failed for array length 2",
9.2f,
NumberUtils.max(new float[] { 6.3f, 9.2f }),
0);
assertEquals(
"max(float[]) failed for float length 5",
10.4f,
NumberUtils.max(new float[] { -10.5f, -5.6f, 0, 5.7f, 10.4f }),
0);
assertEquals(10, NumberUtils.max(new float[] { -10, -5, 0, 5, 10 }), 0.0001f);
assertEquals(10, NumberUtils.max(new float[] { -5, 0, 10, 5, -10 }), 0.0001f);
}
@Test
public void testMinimumLong() {
assertEquals("minimum(long,long,long) 1 failed", 12345L, NumberUtils.min(12345L, 12345L + 1L, 12345L + 2L));
assertEquals("minimum(long,long,long) 2 failed", 12345L, NumberUtils.min(12345L + 1L, 12345L, 12345 + 2L));
assertEquals("minimum(long,long,long) 3 failed", 12345L, NumberUtils.min(12345L + 1L, 12345L + 2L, 12345L));
assertEquals("minimum(long,long,long) 4 failed", 12345L, NumberUtils.min(12345L + 1L, 12345L, 12345L));
assertEquals("minimum(long,long,long) 5 failed", 12345L, NumberUtils.min(12345L, 12345L, 12345L));
}
@Test
public void testMinimumInt() {
assertEquals("minimum(int,int,int) 1 failed", 12345, NumberUtils.min(12345, 12345 + 1, 12345 + 2));
assertEquals("minimum(int,int,int) 2 failed", 12345, NumberUtils.min(12345 + 1, 12345, 12345 + 2));
assertEquals("minimum(int,int,int) 3 failed", 12345, NumberUtils.min(12345 + 1, 12345 + 2, 12345));
assertEquals("minimum(int,int,int) 4 failed", 12345, NumberUtils.min(12345 + 1, 12345, 12345));
assertEquals("minimum(int,int,int) 5 failed", 12345, NumberUtils.min(12345, 12345, 12345));
}
@Test
public void testMinimumShort() {
short low = 1234;
short mid = 1234 + 1;
short high = 1234 + 2;
assertEquals("minimum(short,short,short) 1 failed", low, NumberUtils.min(low, mid, high));
assertEquals("minimum(short,short,short) 1 failed", low, NumberUtils.min(mid, low, high));
assertEquals("minimum(short,short,short) 1 failed", low, NumberUtils.min(mid, high, low));
assertEquals("minimum(short,short,short) 1 failed", low, NumberUtils.min(low, mid, low));
}
@Test
public void testMinimumByte() {
byte low = 123;
byte mid = 123 + 1;
byte high = 123 + 2;
assertEquals("minimum(byte,byte,byte) 1 failed", low, NumberUtils.min(low, mid, high));
assertEquals("minimum(byte,byte,byte) 1 failed", low, NumberUtils.min(mid, low, high));
assertEquals("minimum(byte,byte,byte) 1 failed", low, NumberUtils.min(mid, high, low));
assertEquals("minimum(byte,byte,byte) 1 failed", low, NumberUtils.min(low, mid, low));
}
@Test
public void testMinimumDouble() {
double low = 12.3;
double mid = 12.3 + 1;
double high = 12.3 + 2;
assertEquals(low, NumberUtils.min(low, mid, high), 0.0001);
assertEquals(low, NumberUtils.min(mid, low, high), 0.0001);
assertEquals(low, NumberUtils.min(mid, high, low), 0.0001);
assertEquals(low, NumberUtils.min(low, mid, low), 0.0001);
assertEquals(mid, NumberUtils.min(high, mid, high), 0.0001);
}
@Test
public void testMinimumFloat() {
float low = 12.3f;
float mid = 12.3f + 1;
float high = 12.3f + 2;
assertEquals(low, NumberUtils.min(low, mid, high), 0.0001f);
assertEquals(low, NumberUtils.min(mid, low, high), 0.0001f);
assertEquals(low, NumberUtils.min(mid, high, low), 0.0001f);
assertEquals(low, NumberUtils.min(low, mid, low), 0.0001f);
assertEquals(mid, NumberUtils.min(high, mid, high), 0.0001f);
}
@Test
public void testMaximumLong() {
assertEquals("maximum(long,long,long) 1 failed", 12345L, NumberUtils.max(12345L, 12345L - 1L, 12345L - 2L));
assertEquals("maximum(long,long,long) 2 failed", 12345L, NumberUtils.max(12345L - 1L, 12345L, 12345L - 2L));
assertEquals("maximum(long,long,long) 3 failed", 12345L, NumberUtils.max(12345L - 1L, 12345L - 2L, 12345L));
assertEquals("maximum(long,long,long) 4 failed", 12345L, NumberUtils.max(12345L - 1L, 12345L, 12345L));
assertEquals("maximum(long,long,long) 5 failed", 12345L, NumberUtils.max(12345L, 12345L, 12345L));
}
@Test
public void testMaximumInt() {
assertEquals("maximum(int,int,int) 1 failed", 12345, NumberUtils.max(12345, 12345 - 1, 12345 - 2));
assertEquals("maximum(int,int,int) 2 failed", 12345, NumberUtils.max(12345 - 1, 12345, 12345 - 2));
assertEquals("maximum(int,int,int) 3 failed", 12345, NumberUtils.max(12345 - 1, 12345 - 2, 12345));
assertEquals("maximum(int,int,int) 4 failed", 12345, NumberUtils.max(12345 - 1, 12345, 12345));
assertEquals("maximum(int,int,int) 5 failed", 12345, NumberUtils.max(12345, 12345, 12345));
}
@Test
public void testMaximumShort() {
short low = 1234;
short mid = 1234 + 1;
short high = 1234 + 2;
assertEquals("maximum(short,short,short) 1 failed", high, NumberUtils.max(low, mid, high));
assertEquals("maximum(short,short,short) 1 failed", high, NumberUtils.max(mid, low, high));
assertEquals("maximum(short,short,short) 1 failed", high, NumberUtils.max(mid, high, low));
assertEquals("maximum(short,short,short) 1 failed", high, NumberUtils.max(high, mid, high));
}
@Test
public void testMaximumByte() {
byte low = 123;
byte mid = 123 + 1;
byte high = 123 + 2;
assertEquals("maximum(byte,byte,byte) 1 failed", high, NumberUtils.max(low, mid, high));
assertEquals("maximum(byte,byte,byte) 1 failed", high, NumberUtils.max(mid, low, high));
assertEquals("maximum(byte,byte,byte) 1 failed", high, NumberUtils.max(mid, high, low));
assertEquals("maximum(byte,byte,byte) 1 failed", high, NumberUtils.max(high, mid, high));
}
@Test
public void testMaximumDouble() {
double low = 12.3;
double mid = 12.3 + 1;
double high = 12.3 + 2;
assertEquals(high, NumberUtils.max(low, mid, high), 0.0001);
assertEquals(high, NumberUtils.max(mid, low, high), 0.0001);
assertEquals(high, NumberUtils.max(mid, high, low), 0.0001);
assertEquals(mid, NumberUtils.max(low, mid, low), 0.0001);
assertEquals(high, NumberUtils.max(high, mid, high), 0.0001);
}
@Test
public void testMaximumFloat() {
float low = 12.3f;
float mid = 12.3f + 1;
float high = 12.3f + 2;
assertEquals(high, NumberUtils.max(low, mid, high), 0.0001f);
assertEquals(high, NumberUtils.max(mid, low, high), 0.0001f);
assertEquals(high, NumberUtils.max(mid, high, low), 0.0001f);
assertEquals(mid, NumberUtils.max(low, mid, low), 0.0001f);
assertEquals(high, NumberUtils.max(high, mid, high), 0.0001f);
}
// Testing JDK against old Lang functionality
@Test
public void testCompareDouble() {
assertTrue(Double.compare(Double.NaN, Double.NaN) == 0);
assertTrue(Double.compare(Double.NaN, Double.POSITIVE_INFINITY) == +1);
assertTrue(Double.compare(Double.NaN, Double.MAX_VALUE) == +1);
assertTrue(Double.compare(Double.NaN, 1.2d) == +1);
assertTrue(Double.compare(Double.NaN, 0.0d) == +1);
assertTrue(Double.compare(Double.NaN, -0.0d) == +1);
assertTrue(Double.compare(Double.NaN, -1.2d) == +1);
assertTrue(Double.compare(Double.NaN, -Double.MAX_VALUE) == +1);
assertTrue(Double.compare(Double.NaN, Double.NEGATIVE_INFINITY) == +1);
assertTrue(Double.compare(Double.POSITIVE_INFINITY, Double.NaN) == -1);
assertTrue(Double.compare(Double.POSITIVE_INFINITY, Double.POSITIVE_INFINITY) == 0);
assertTrue(Double.compare(Double.POSITIVE_INFINITY, Double.MAX_VALUE) == +1);
assertTrue(Double.compare(Double.POSITIVE_INFINITY, 1.2d) == +1);
assertTrue(Double.compare(Double.POSITIVE_INFINITY, 0.0d) == +1);
assertTrue(Double.compare(Double.POSITIVE_INFINITY, -0.0d) == +1);
assertTrue(Double.compare(Double.POSITIVE_INFINITY, -1.2d) == +1);
assertTrue(Double.compare(Double.POSITIVE_INFINITY, -Double.MAX_VALUE) == +1);
assertTrue(Double.compare(Double.POSITIVE_INFINITY, Double.NEGATIVE_INFINITY) == +1);
assertTrue(Double.compare(Double.MAX_VALUE, Double.NaN) == -1);
assertTrue(Double.compare(Double.MAX_VALUE, Double.POSITIVE_INFINITY) == -1);
assertTrue(Double.compare(Double.MAX_VALUE, Double.MAX_VALUE) == 0);
assertTrue(Double.compare(Double.MAX_VALUE, 1.2d) == +1);
assertTrue(Double.compare(Double.MAX_VALUE, 0.0d) == +1);
assertTrue(Double.compare(Double.MAX_VALUE, -0.0d) == +1);
assertTrue(Double.compare(Double.MAX_VALUE, -1.2d) == +1);
assertTrue(Double.compare(Double.MAX_VALUE, -Double.MAX_VALUE) == +1);
assertTrue(Double.compare(Double.MAX_VALUE, Double.NEGATIVE_INFINITY) == +1);
assertTrue(Double.compare(1.2d, Double.NaN) == -1);
assertTrue(Double.compare(1.2d, Double.POSITIVE_INFINITY) == -1);
assertTrue(Double.compare(1.2d, Double.MAX_VALUE) == -1);
assertTrue(Double.compare(1.2d, 1.2d) == 0);
assertTrue(Double.compare(1.2d, 0.0d) == +1);
assertTrue(Double.compare(1.2d, -0.0d) == +1);
assertTrue(Double.compare(1.2d, -1.2d) == +1);
assertTrue(Double.compare(1.2d, -Double.MAX_VALUE) == +1);
assertTrue(Double.compare(1.2d, Double.NEGATIVE_INFINITY) == +1);
assertTrue(Double.compare(0.0d, Double.NaN) == -1);
assertTrue(Double.compare(0.0d, Double.POSITIVE_INFINITY) == -1);
assertTrue(Double.compare(0.0d, Double.MAX_VALUE) == -1);
assertTrue(Double.compare(0.0d, 1.2d) == -1);
assertTrue(Double.compare(0.0d, 0.0d) == 0);
assertTrue(Double.compare(0.0d, -0.0d) == +1);
assertTrue(Double.compare(0.0d, -1.2d) == +1);
assertTrue(Double.compare(0.0d, -Double.MAX_VALUE) == +1);
assertTrue(Double.compare(0.0d, Double.NEGATIVE_INFINITY) == +1);
assertTrue(Double.compare(-0.0d, Double.NaN) == -1);
assertTrue(Double.compare(-0.0d, Double.POSITIVE_INFINITY) == -1);
assertTrue(Double.compare(-0.0d, Double.MAX_VALUE) == -1);
assertTrue(Double.compare(-0.0d, 1.2d) == -1);
assertTrue(Double.compare(-0.0d, 0.0d) == -1);
assertTrue(Double.compare(-0.0d, -0.0d) == 0);
assertTrue(Double.compare(-0.0d, -1.2d) == +1);
assertTrue(Double.compare(-0.0d, -Double.MAX_VALUE) == +1);
assertTrue(Double.compare(-0.0d, Double.NEGATIVE_INFINITY) == +1);
assertTrue(Double.compare(-1.2d, Double.NaN) == -1);
assertTrue(Double.compare(-1.2d, Double.POSITIVE_INFINITY) == -1);
assertTrue(Double.compare(-1.2d, Double.MAX_VALUE) == -1);
assertTrue(Double.compare(-1.2d, 1.2d) == -1);
assertTrue(Double.compare(-1.2d, 0.0d) == -1);
assertTrue(Double.compare(-1.2d, -0.0d) == -1);
assertTrue(Double.compare(-1.2d, -1.2d) == 0);
assertTrue(Double.compare(-1.2d, -Double.MAX_VALUE) == +1);
assertTrue(Double.compare(-1.2d, Double.NEGATIVE_INFINITY) == +1);
assertTrue(Double.compare(-Double.MAX_VALUE, Double.NaN) == -1);
assertTrue(Double.compare(-Double.MAX_VALUE, Double.POSITIVE_INFINITY) == -1);
assertTrue(Double.compare(-Double.MAX_VALUE, Double.MAX_VALUE) == -1);
assertTrue(Double.compare(-Double.MAX_VALUE, 1.2d) == -1);
assertTrue(Double.compare(-Double.MAX_VALUE, 0.0d) == -1);
assertTrue(Double.compare(-Double.MAX_VALUE, -0.0d) == -1);
assertTrue(Double.compare(-Double.MAX_VALUE, -1.2d) == -1);
assertTrue(Double.compare(-Double.MAX_VALUE, -Double.MAX_VALUE) == 0);
assertTrue(Double.compare(-Double.MAX_VALUE, Double.NEGATIVE_INFINITY) == +1);
assertTrue(Double.compare(Double.NEGATIVE_INFINITY, Double.NaN) == -1);
assertTrue(Double.compare(Double.NEGATIVE_INFINITY, Double.POSITIVE_INFINITY) == -1);
assertTrue(Double.compare(Double.NEGATIVE_INFINITY, Double.MAX_VALUE) == -1);
assertTrue(Double.compare(Double.NEGATIVE_INFINITY, 1.2d) == -1);
assertTrue(Double.compare(Double.NEGATIVE_INFINITY, 0.0d) == -1);
assertTrue(Double.compare(Double.NEGATIVE_INFINITY, -0.0d) == -1);
assertTrue(Double.compare(Double.NEGATIVE_INFINITY, -1.2d) == -1);
assertTrue(Double.compare(Double.NEGATIVE_INFINITY, -Double.MAX_VALUE) == -1);
assertTrue(Double.compare(Double.NEGATIVE_INFINITY, Double.NEGATIVE_INFINITY) == 0);
}
@Test
public void testCompareFloat() {
assertTrue(Float.compare(Float.NaN, Float.NaN) == 0);
assertTrue(Float.compare(Float.NaN, Float.POSITIVE_INFINITY) == +1);
assertTrue(Float.compare(Float.NaN, Float.MAX_VALUE) == +1);
assertTrue(Float.compare(Float.NaN, 1.2f) == +1);
assertTrue(Float.compare(Float.NaN, 0.0f) == +1);
assertTrue(Float.compare(Float.NaN, -0.0f) == +1);
assertTrue(Float.compare(Float.NaN, -1.2f) == +1);
assertTrue(Float.compare(Float.NaN, -Float.MAX_VALUE) == +1);
assertTrue(Float.compare(Float.NaN, Float.NEGATIVE_INFINITY) == +1);
assertTrue(Float.compare(Float.POSITIVE_INFINITY, Float.NaN) == -1);
assertTrue(Float.compare(Float.POSITIVE_INFINITY, Float.POSITIVE_INFINITY) == 0);
assertTrue(Float.compare(Float.POSITIVE_INFINITY, Float.MAX_VALUE) == +1);
assertTrue(Float.compare(Float.POSITIVE_INFINITY, 1.2f) == +1);
assertTrue(Float.compare(Float.POSITIVE_INFINITY, 0.0f) == +1);
assertTrue(Float.compare(Float.POSITIVE_INFINITY, -0.0f) == +1);
assertTrue(Float.compare(Float.POSITIVE_INFINITY, -1.2f) == +1);
assertTrue(Float.compare(Float.POSITIVE_INFINITY, -Float.MAX_VALUE) == +1);
assertTrue(Float.compare(Float.POSITIVE_INFINITY, Float.NEGATIVE_INFINITY) == +1);
assertTrue(Float.compare(Float.MAX_VALUE, Float.NaN) == -1);
assertTrue(Float.compare(Float.MAX_VALUE, Float.POSITIVE_INFINITY) == -1);
assertTrue(Float.compare(Float.MAX_VALUE, Float.MAX_VALUE) == 0);
assertTrue(Float.compare(Float.MAX_VALUE, 1.2f) == +1);
assertTrue(Float.compare(Float.MAX_VALUE, 0.0f) == +1);
assertTrue(Float.compare(Float.MAX_VALUE, -0.0f) == +1);
assertTrue(Float.compare(Float.MAX_VALUE, -1.2f) == +1);
assertTrue(Float.compare(Float.MAX_VALUE, -Float.MAX_VALUE) == +1);
assertTrue(Float.compare(Float.MAX_VALUE, Float.NEGATIVE_INFINITY) == +1);
assertTrue(Float.compare(1.2f, Float.NaN) == -1);
assertTrue(Float.compare(1.2f, Float.POSITIVE_INFINITY) == -1);
assertTrue(Float.compare(1.2f, Float.MAX_VALUE) == -1);
assertTrue(Float.compare(1.2f, 1.2f) == 0);
assertTrue(Float.compare(1.2f, 0.0f) == +1);
assertTrue(Float.compare(1.2f, -0.0f) == +1);
assertTrue(Float.compare(1.2f, -1.2f) == +1);
assertTrue(Float.compare(1.2f, -Float.MAX_VALUE) == +1);
assertTrue(Float.compare(1.2f, Float.NEGATIVE_INFINITY) == +1);
assertTrue(Float.compare(0.0f, Float.NaN) == -1);
assertTrue(Float.compare(0.0f, Float.POSITIVE_INFINITY) == -1);
assertTrue(Float.compare(0.0f, Float.MAX_VALUE) == -1);
assertTrue(Float.compare(0.0f, 1.2f) == -1);
assertTrue(Float.compare(0.0f, 0.0f) == 0);
assertTrue(Float.compare(0.0f, -0.0f) == +1);
assertTrue(Float.compare(0.0f, -1.2f) == +1);
assertTrue(Float.compare(0.0f, -Float.MAX_VALUE) == +1);
assertTrue(Float.compare(0.0f, Float.NEGATIVE_INFINITY) == +1);
assertTrue(Float.compare(-0.0f, Float.NaN) == -1);
assertTrue(Float.compare(-0.0f, Float.POSITIVE_INFINITY) == -1);
assertTrue(Float.compare(-0.0f, Float.MAX_VALUE) == -1);
assertTrue(Float.compare(-0.0f, 1.2f) == -1);
assertTrue(Float.compare(-0.0f, 0.0f) == -1);
assertTrue(Float.compare(-0.0f, -0.0f) == 0);
assertTrue(Float.compare(-0.0f, -1.2f) == +1);
assertTrue(Float.compare(-0.0f, -Float.MAX_VALUE) == +1);
assertTrue(Float.compare(-0.0f, Float.NEGATIVE_INFINITY) == +1);
assertTrue(Float.compare(-1.2f, Float.NaN) == -1);
assertTrue(Float.compare(-1.2f, Float.POSITIVE_INFINITY) == -1);
assertTrue(Float.compare(-1.2f, Float.MAX_VALUE) == -1);
assertTrue(Float.compare(-1.2f, 1.2f) == -1);
assertTrue(Float.compare(-1.2f, 0.0f) == -1);
assertTrue(Float.compare(-1.2f, -0.0f) == -1);
assertTrue(Float.compare(-1.2f, -1.2f) == 0);
assertTrue(Float.compare(-1.2f, -Float.MAX_VALUE) == +1);
assertTrue(Float.compare(-1.2f, Float.NEGATIVE_INFINITY) == +1);
assertTrue(Float.compare(-Float.MAX_VALUE, Float.NaN) == -1);
assertTrue(Float.compare(-Float.MAX_VALUE, Float.POSITIVE_INFINITY) == -1);
assertTrue(Float.compare(-Float.MAX_VALUE, Float.MAX_VALUE) == -1);
assertTrue(Float.compare(-Float.MAX_VALUE, 1.2f) == -1);
assertTrue(Float.compare(-Float.MAX_VALUE, 0.0f) == -1);
assertTrue(Float.compare(-Float.MAX_VALUE, -0.0f) == -1);
assertTrue(Float.compare(-Float.MAX_VALUE, -1.2f) == -1);
assertTrue(Float.compare(-Float.MAX_VALUE, -Float.MAX_VALUE) == 0);
assertTrue(Float.compare(-Float.MAX_VALUE, Float.NEGATIVE_INFINITY) == +1);
assertTrue(Float.compare(Float.NEGATIVE_INFINITY, Float.NaN) == -1);
assertTrue(Float.compare(Float.NEGATIVE_INFINITY, Float.POSITIVE_INFINITY) == -1);
assertTrue(Float.compare(Float.NEGATIVE_INFINITY, Float.MAX_VALUE) == -1);
assertTrue(Float.compare(Float.NEGATIVE_INFINITY, 1.2f) == -1);
assertTrue(Float.compare(Float.NEGATIVE_INFINITY, 0.0f) == -1);
assertTrue(Float.compare(Float.NEGATIVE_INFINITY, -0.0f) == -1);
assertTrue(Float.compare(Float.NEGATIVE_INFINITY, -1.2f) == -1);
assertTrue(Float.compare(Float.NEGATIVE_INFINITY, -Float.MAX_VALUE) == -1);
assertTrue(Float.compare(Float.NEGATIVE_INFINITY, Float.NEGATIVE_INFINITY) == 0);
}
@Test
public void testIsDigits() {
assertFalse("isDigits(null) failed", NumberUtils.isDigits(null));
assertFalse("isDigits('') failed", NumberUtils.isDigits(""));
assertTrue("isDigits(String) failed", NumberUtils.isDigits("12345"));
assertFalse("isDigits(String) neg 1 failed", NumberUtils.isDigits("1234.5"));
assertFalse("isDigits(String) neg 3 failed", NumberUtils.isDigits("1ab"));
assertFalse("isDigits(String) neg 4 failed", NumberUtils.isDigits("abc"));
}
/**
* Tests isNumber(String) and tests that createNumber(String) returns
* a valid number iff isNumber(String) returns false.
*/
@Test
public void testIsNumber() {
String val = "12345";
assertTrue("isNumber(String) 1 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 1 failed", checkCreateNumber(val));
val = "1234.5";
assertTrue("isNumber(String) 2 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 2 failed", checkCreateNumber(val));
val = ".12345";
assertTrue("isNumber(String) 3 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 3 failed", checkCreateNumber(val));
val = "1234E5";
assertTrue("isNumber(String) 4 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 4 failed", checkCreateNumber(val));
val = "1234E+5";
assertTrue("isNumber(String) 5 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 5 failed", checkCreateNumber(val));
val = "1234E-5";
assertTrue("isNumber(String) 6 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 6 failed", checkCreateNumber(val));
val = "123.4E5";
assertTrue("isNumber(String) 7 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 7 failed", checkCreateNumber(val));
val = "-1234";
assertTrue("isNumber(String) 8 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 8 failed", checkCreateNumber(val));
val = "-1234.5";
assertTrue("isNumber(String) 9 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 9 failed", checkCreateNumber(val));
val = "-.12345";
assertTrue("isNumber(String) 10 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 10 failed", checkCreateNumber(val));
val = "-1234E5";
assertTrue("isNumber(String) 11 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 11 failed", checkCreateNumber(val));
val = "0";
assertTrue("isNumber(String) 12 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 12 failed", checkCreateNumber(val));
val = "-0";
assertTrue("isNumber(String) 13 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 13 failed", checkCreateNumber(val));
val = "01234";
assertTrue("isNumber(String) 14 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 14 failed", checkCreateNumber(val));
val = "-01234";
assertTrue("isNumber(String) 15 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 15 failed", checkCreateNumber(val));
val = "0xABC123";
assertTrue("isNumber(String) 16 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 16 failed", checkCreateNumber(val));
val = "0x0";
assertTrue("isNumber(String) 17 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 17 failed", checkCreateNumber(val));
val = "123.4E21D";
assertTrue("isNumber(String) 19 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 19 failed", checkCreateNumber(val));
val = "-221.23F";
assertTrue("isNumber(String) 20 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 20 failed", checkCreateNumber(val));
val = "22338L";
assertTrue("isNumber(String) 21 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 21 failed", checkCreateNumber(val));
val = null;
assertTrue("isNumber(String) 1 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 1 Neg failed", !checkCreateNumber(val));
val = "";
assertTrue("isNumber(String) 2 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 2 Neg failed", !checkCreateNumber(val));
val = "--2.3";
assertTrue("isNumber(String) 3 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 3 Neg failed", !checkCreateNumber(val));
val = ".12.3";
assertTrue("isNumber(String) 4 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 4 Neg failed", !checkCreateNumber(val));
val = "-123E";
assertTrue("isNumber(String) 5 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 5 Neg failed", !checkCreateNumber(val));
val = "-123E+-212";
assertTrue("isNumber(String) 6 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 6 Neg failed", !checkCreateNumber(val));
val = "-123E2.12";
assertTrue("isNumber(String) 7 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 7 Neg failed", !checkCreateNumber(val));
val = "0xGF";
assertTrue("isNumber(String) 8 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 8 Neg failed", !checkCreateNumber(val));
val = "0xFAE-1";
assertTrue("isNumber(String) 9 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 9 Neg failed", !checkCreateNumber(val));
val = ".";
assertTrue("isNumber(String) 10 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 10 Neg failed", !checkCreateNumber(val));
val = "-0ABC123";
assertTrue("isNumber(String) 11 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 11 Neg failed", !checkCreateNumber(val));
val = "123.4E-D";
assertTrue("isNumber(String) 12 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 12 Neg failed", !checkCreateNumber(val));
val = "123.4ED";
assertTrue("isNumber(String) 13 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 13 Neg failed", !checkCreateNumber(val));
val = "1234E5l";
assertTrue("isNumber(String) 14 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 14 Neg failed", !checkCreateNumber(val));
val = "11a";
assertTrue("isNumber(String) 15 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 15 Neg failed", !checkCreateNumber(val));
val = "1a";
assertTrue("isNumber(String) 16 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 16 Neg failed", !checkCreateNumber(val));
val = "a";
assertTrue("isNumber(String) 17 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 17 Neg failed", !checkCreateNumber(val));
val = "11g";
assertTrue("isNumber(String) 18 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 18 Neg failed", !checkCreateNumber(val));
val = "11z";
assertTrue("isNumber(String) 19 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 19 Neg failed", !checkCreateNumber(val));
val = "11def";
assertTrue("isNumber(String) 20 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 20 Neg failed", !checkCreateNumber(val));
val = "11d11";
assertTrue("isNumber(String) 21 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 21 Neg failed", !checkCreateNumber(val));
val = "11 11";
assertTrue("isNumber(String) 22 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 22 Neg failed", !checkCreateNumber(val));
val = " 1111";
assertTrue("isNumber(String) 23 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 23 Neg failed", !checkCreateNumber(val));
val = "1111 ";
assertTrue("isNumber(String) 24 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 24 Neg failed", !checkCreateNumber(val));
// LANG-521
val = "2.";
assertTrue("isNumber(String) LANG-521 failed", NumberUtils.isNumber(val));
// LANG-664
val = "1.1L";
assertFalse("isNumber(String) LANG-664 failed", NumberUtils.isNumber(val));
}
private boolean checkCreateNumber(String val) {
try {
Object obj = NumberUtils.createNumber(val);
if (obj == null) {
return false;
}
return true;
} catch (NumberFormatException e) {
return false;
}
}
@SuppressWarnings("cast") // suppress instanceof warning check
@Test
public void testConstants() {
assertTrue(NumberUtils.LONG_ZERO instanceof Long);
assertTrue(NumberUtils.LONG_ONE instanceof Long);
assertTrue(NumberUtils.LONG_MINUS_ONE instanceof Long);
assertTrue(NumberUtils.INTEGER_ZERO instanceof Integer);
assertTrue(NumberUtils.INTEGER_ONE instanceof Integer);
assertTrue(NumberUtils.INTEGER_MINUS_ONE instanceof Integer);
assertTrue(NumberUtils.SHORT_ZERO instanceof Short);
assertTrue(NumberUtils.SHORT_ONE instanceof Short);
assertTrue(NumberUtils.SHORT_MINUS_ONE instanceof Short);
assertTrue(NumberUtils.BYTE_ZERO instanceof Byte);
assertTrue(NumberUtils.BYTE_ONE instanceof Byte);
assertTrue(NumberUtils.BYTE_MINUS_ONE instanceof Byte);
assertTrue(NumberUtils.DOUBLE_ZERO instanceof Double);
assertTrue(NumberUtils.DOUBLE_ONE instanceof Double);
assertTrue(NumberUtils.DOUBLE_MINUS_ONE instanceof Double);
assertTrue(NumberUtils.FLOAT_ZERO instanceof Float);
assertTrue(NumberUtils.FLOAT_ONE instanceof Float);
assertTrue(NumberUtils.FLOAT_MINUS_ONE instanceof Float);
assertTrue(NumberUtils.LONG_ZERO.longValue() == 0);
assertTrue(NumberUtils.LONG_ONE.longValue() == 1);
assertTrue(NumberUtils.LONG_MINUS_ONE.longValue() == -1);
assertTrue(NumberUtils.INTEGER_ZERO.intValue() == 0);
assertTrue(NumberUtils.INTEGER_ONE.intValue() == 1);
assertTrue(NumberUtils.INTEGER_MINUS_ONE.intValue() == -1);
assertTrue(NumberUtils.SHORT_ZERO.shortValue() == 0);
assertTrue(NumberUtils.SHORT_ONE.shortValue() == 1);
assertTrue(NumberUtils.SHORT_MINUS_ONE.shortValue() == -1);
assertTrue(NumberUtils.BYTE_ZERO.byteValue() == 0);
assertTrue(NumberUtils.BYTE_ONE.byteValue() == 1);
assertTrue(NumberUtils.BYTE_MINUS_ONE.byteValue() == -1);
assertTrue(NumberUtils.DOUBLE_ZERO.doubleValue() == 0.0d);
assertTrue(NumberUtils.DOUBLE_ONE.doubleValue() == 1.0d);
assertTrue(NumberUtils.DOUBLE_MINUS_ONE.doubleValue() == -1.0d);
assertTrue(NumberUtils.FLOAT_ZERO.floatValue() == 0.0f);
assertTrue(NumberUtils.FLOAT_ONE.floatValue() == 1.0f);
assertTrue(NumberUtils.FLOAT_MINUS_ONE.floatValue() == -1.0f);
}
@Test
public void testLang300() {
NumberUtils.createNumber("-1l");
NumberUtils.createNumber("01l");
NumberUtils.createNumber("1l");
}
@Test
public void testLang381() {
assertTrue(Double.isNaN(NumberUtils.min(1.2, 2.5, Double.NaN)));
assertTrue(Double.isNaN(NumberUtils.max(1.2, 2.5, Double.NaN)));
assertTrue(Float.isNaN(NumberUtils.min(1.2f, 2.5f, Float.NaN)));
assertTrue(Float.isNaN(NumberUtils.max(1.2f, 2.5f, Float.NaN)));
double[] a = new double[] { 1.2, Double.NaN, 3.7, 27.0, 42.0, Double.NaN };
assertTrue(Double.isNaN(NumberUtils.max(a)));
assertTrue(Double.isNaN(NumberUtils.min(a)));
double[] b = new double[] { Double.NaN, 1.2, Double.NaN, 3.7, 27.0, 42.0, Double.NaN };
assertTrue(Double.isNaN(NumberUtils.max(b)));
assertTrue(Double.isNaN(NumberUtils.min(b)));
float[] aF = new float[] { 1.2f, Float.NaN, 3.7f, 27.0f, 42.0f, Float.NaN };
assertTrue(Float.isNaN(NumberUtils.max(aF)));
float[] bF = new float[] { Float.NaN, 1.2f, Float.NaN, 3.7f, 27.0f, 42.0f, Float.NaN };
assertTrue(Float.isNaN(NumberUtils.max(bF)));
}
} | // You are a professional Java test case writer, please create a test case named `testCreateNumber` for the issue `Lang-LANG-746`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Lang-LANG-746
//
// ## Issue-Title:
// NumberUtils does not handle upper-case hex: 0X and -0X
//
// ## Issue-Description:
//
// NumberUtils.createNumber() should work equally for 0x1234 and 0X1234; currently 0X1234 generates a NumberFormatException
//
//
// Integer.decode() handles both upper and lower case hex.
//
//
//
//
//
@Test
public void testCreateNumber() {
| 228 | 16 | 180 | src/test/java/org/apache/commons/lang3/math/NumberUtilsTest.java | src/test/java | ```markdown
## Issue-ID: Lang-LANG-746
## Issue-Title:
NumberUtils does not handle upper-case hex: 0X and -0X
## Issue-Description:
NumberUtils.createNumber() should work equally for 0x1234 and 0X1234; currently 0X1234 generates a NumberFormatException
Integer.decode() handles both upper and lower case hex.
```
You are a professional Java test case writer, please create a test case named `testCreateNumber` for the issue `Lang-LANG-746`, utilizing the provided issue report information and the following function signature.
```java
@Test
public void testCreateNumber() {
```
| 180 | [
"org.apache.commons.lang3.math.NumberUtils"
] | 8952c043dae764b33a089f56fd003e5a32d21949d61d9c71adfe85c8e94df5d4 | @Test
public void testCreateNumber() | // You are a professional Java test case writer, please create a test case named `testCreateNumber` for the issue `Lang-LANG-746`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Lang-LANG-746
//
// ## Issue-Title:
// NumberUtils does not handle upper-case hex: 0X and -0X
//
// ## Issue-Description:
//
// NumberUtils.createNumber() should work equally for 0x1234 and 0X1234; currently 0X1234 generates a NumberFormatException
//
//
// Integer.decode() handles both upper and lower case hex.
//
//
//
//
//
| Lang | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.lang3.math;
import static org.apache.commons.lang3.JavaVersion.JAVA_1_3;
import static org.junit.Assert.*;
import java.lang.reflect.Constructor;
import java.lang.reflect.Modifier;
import java.math.BigDecimal;
import java.math.BigInteger;
import org.apache.commons.lang3.SystemUtils;
import org.junit.Test;
/**
* Unit tests {@link org.apache.commons.lang3.math.NumberUtils}.
*
* @version $Id$
*/
public class NumberUtilsTest {
//-----------------------------------------------------------------------
@Test
public void testConstructor() {
assertNotNull(new NumberUtils());
Constructor<?>[] cons = NumberUtils.class.getDeclaredConstructors();
assertEquals(1, cons.length);
assertTrue(Modifier.isPublic(cons[0].getModifiers()));
assertTrue(Modifier.isPublic(NumberUtils.class.getModifiers()));
assertFalse(Modifier.isFinal(NumberUtils.class.getModifiers()));
}
//---------------------------------------------------------------------
/**
* Test for {@link NumberUtils#toInt(String)}.
*/
@Test
public void testToIntString() {
assertTrue("toInt(String) 1 failed", NumberUtils.toInt("12345") == 12345);
assertTrue("toInt(String) 2 failed", NumberUtils.toInt("abc") == 0);
assertTrue("toInt(empty) failed", NumberUtils.toInt("") == 0);
assertTrue("toInt(null) failed", NumberUtils.toInt(null) == 0);
}
/**
* Test for {@link NumberUtils#toInt(String, int)}.
*/
@Test
public void testToIntStringI() {
assertTrue("toInt(String,int) 1 failed", NumberUtils.toInt("12345", 5) == 12345);
assertTrue("toInt(String,int) 2 failed", NumberUtils.toInt("1234.5", 5) == 5);
}
/**
* Test for {@link NumberUtils#toLong(String)}.
*/
@Test
public void testToLongString() {
assertTrue("toLong(String) 1 failed", NumberUtils.toLong("12345") == 12345l);
assertTrue("toLong(String) 2 failed", NumberUtils.toLong("abc") == 0l);
assertTrue("toLong(String) 3 failed", NumberUtils.toLong("1L") == 0l);
assertTrue("toLong(String) 4 failed", NumberUtils.toLong("1l") == 0l);
assertTrue("toLong(Long.MAX_VALUE) failed", NumberUtils.toLong(Long.MAX_VALUE+"") == Long.MAX_VALUE);
assertTrue("toLong(Long.MIN_VALUE) failed", NumberUtils.toLong(Long.MIN_VALUE+"") == Long.MIN_VALUE);
assertTrue("toLong(empty) failed", NumberUtils.toLong("") == 0l);
assertTrue("toLong(null) failed", NumberUtils.toLong(null) == 0l);
}
/**
* Test for {@link NumberUtils#toLong(String, long)}.
*/
@Test
public void testToLongStringL() {
assertTrue("toLong(String,long) 1 failed", NumberUtils.toLong("12345", 5l) == 12345l);
assertTrue("toLong(String,long) 2 failed", NumberUtils.toLong("1234.5", 5l) == 5l);
}
/**
* Test for {@link NumberUtils#toFloat(String)}.
*/
@Test
public void testToFloatString() {
assertTrue("toFloat(String) 1 failed", NumberUtils.toFloat("-1.2345") == -1.2345f);
assertTrue("toFloat(String) 2 failed", NumberUtils.toFloat("1.2345") == 1.2345f);
assertTrue("toFloat(String) 3 failed", NumberUtils.toFloat("abc") == 0.0f);
assertTrue("toFloat(Float.MAX_VALUE) failed", NumberUtils.toFloat(Float.MAX_VALUE+"") == Float.MAX_VALUE);
assertTrue("toFloat(Float.MIN_VALUE) failed", NumberUtils.toFloat(Float.MIN_VALUE+"") == Float.MIN_VALUE);
assertTrue("toFloat(empty) failed", NumberUtils.toFloat("") == 0.0f);
assertTrue("toFloat(null) failed", NumberUtils.toFloat(null) == 0.0f);
}
/**
* Test for {@link NumberUtils#toFloat(String, float)}.
*/
@Test
public void testToFloatStringF() {
assertTrue("toFloat(String,int) 1 failed", NumberUtils.toFloat("1.2345", 5.1f) == 1.2345f);
assertTrue("toFloat(String,int) 2 failed", NumberUtils.toFloat("a", 5.0f) == 5.0f);
}
/**
* Test for {@link NumberUtils#toDouble(String)}.
*/
@Test
public void testStringToDoubleString() {
assertTrue("toDouble(String) 1 failed", NumberUtils.toDouble("-1.2345") == -1.2345d);
assertTrue("toDouble(String) 2 failed", NumberUtils.toDouble("1.2345") == 1.2345d);
assertTrue("toDouble(String) 3 failed", NumberUtils.toDouble("abc") == 0.0d);
assertTrue("toDouble(Double.MAX_VALUE) failed", NumberUtils.toDouble(Double.MAX_VALUE+"") == Double.MAX_VALUE);
assertTrue("toDouble(Double.MIN_VALUE) failed", NumberUtils.toDouble(Double.MIN_VALUE+"") == Double.MIN_VALUE);
assertTrue("toDouble(empty) failed", NumberUtils.toDouble("") == 0.0d);
assertTrue("toDouble(null) failed", NumberUtils.toDouble(null) == 0.0d);
}
/**
* Test for {@link NumberUtils#toDouble(String, double)}.
*/
@Test
public void testStringToDoubleStringD() {
assertTrue("toDouble(String,int) 1 failed", NumberUtils.toDouble("1.2345", 5.1d) == 1.2345d);
assertTrue("toDouble(String,int) 2 failed", NumberUtils.toDouble("a", 5.0d) == 5.0d);
}
/**
* Test for {@link NumberUtils#toByte(String)}.
*/
@Test
public void testToByteString() {
assertTrue("toByte(String) 1 failed", NumberUtils.toByte("123") == 123);
assertTrue("toByte(String) 2 failed", NumberUtils.toByte("abc") == 0);
assertTrue("toByte(empty) failed", NumberUtils.toByte("") == 0);
assertTrue("toByte(null) failed", NumberUtils.toByte(null) == 0);
}
/**
* Test for {@link NumberUtils#toByte(String, byte)}.
*/
@Test
public void testToByteStringI() {
assertTrue("toByte(String,byte) 1 failed", NumberUtils.toByte("123", (byte) 5) == 123);
assertTrue("toByte(String,byte) 2 failed", NumberUtils.toByte("12.3", (byte) 5) == 5);
}
/**
* Test for {@link NumberUtils#toShort(String)}.
*/
@Test
public void testToShortString() {
assertTrue("toShort(String) 1 failed", NumberUtils.toShort("12345") == 12345);
assertTrue("toShort(String) 2 failed", NumberUtils.toShort("abc") == 0);
assertTrue("toShort(empty) failed", NumberUtils.toShort("") == 0);
assertTrue("toShort(null) failed", NumberUtils.toShort(null) == 0);
}
/**
* Test for {@link NumberUtils#toShort(String, short)}.
*/
@Test
public void testToShortStringI() {
assertTrue("toShort(String,short) 1 failed", NumberUtils.toShort("12345", (short) 5) == 12345);
assertTrue("toShort(String,short) 2 failed", NumberUtils.toShort("1234.5", (short) 5) == 5);
}
@Test
public void testCreateNumber() {
// a lot of things can go wrong
assertEquals("createNumber(String) 1 failed", Float.valueOf("1234.5"), NumberUtils.createNumber("1234.5"));
assertEquals("createNumber(String) 2 failed", Integer.valueOf("12345"), NumberUtils.createNumber("12345"));
assertEquals("createNumber(String) 3 failed", Double.valueOf("1234.5"), NumberUtils.createNumber("1234.5D"));
assertEquals("createNumber(String) 3 failed", Double.valueOf("1234.5"), NumberUtils.createNumber("1234.5d"));
assertEquals("createNumber(String) 4 failed", Float.valueOf("1234.5"), NumberUtils.createNumber("1234.5F"));
assertEquals("createNumber(String) 4 failed", Float.valueOf("1234.5"), NumberUtils.createNumber("1234.5f"));
assertEquals("createNumber(String) 5 failed", Long.valueOf(Integer.MAX_VALUE + 1L), NumberUtils.createNumber(""
+ (Integer.MAX_VALUE + 1L)));
assertEquals("createNumber(String) 6 failed", Long.valueOf(12345), NumberUtils.createNumber("12345L"));
assertEquals("createNumber(String) 6 failed", Long.valueOf(12345), NumberUtils.createNumber("12345l"));
assertEquals("createNumber(String) 7 failed", Float.valueOf("-1234.5"), NumberUtils.createNumber("-1234.5"));
assertEquals("createNumber(String) 8 failed", Integer.valueOf("-12345"), NumberUtils.createNumber("-12345"));
assertTrue("createNumber(String) 9a failed", 0xFADE == NumberUtils.createNumber("0xFADE").intValue());
assertTrue("createNumber(String) 9b failed", 0xFADE == NumberUtils.createNumber("0Xfade").intValue());
assertTrue("createNumber(String) 10a failed", -0xFADE == NumberUtils.createNumber("-0xFADE").intValue());
assertTrue("createNumber(String) 10b failed", -0xFADE == NumberUtils.createNumber("-0Xfade").intValue());
assertEquals("createNumber(String) 11 failed", Double.valueOf("1.1E200"), NumberUtils.createNumber("1.1E200"));
assertEquals("createNumber(String) 12 failed", Float.valueOf("1.1E20"), NumberUtils.createNumber("1.1E20"));
assertEquals("createNumber(String) 13 failed", Double.valueOf("-1.1E200"), NumberUtils.createNumber("-1.1E200"));
assertEquals("createNumber(String) 14 failed", Double.valueOf("1.1E-200"), NumberUtils.createNumber("1.1E-200"));
assertEquals("createNumber(null) failed", null, NumberUtils.createNumber(null));
assertEquals("createNumber(String) failed", new BigInteger("12345678901234567890"), NumberUtils
.createNumber("12345678901234567890L"));
// jdk 1.2 doesn't support this. unsure about jdk 1.2.2
if (SystemUtils.isJavaVersionAtLeast(JAVA_1_3)) {
assertEquals("createNumber(String) 15 failed", new BigDecimal("1.1E-700"), NumberUtils
.createNumber("1.1E-700F"));
}
assertEquals("createNumber(String) 16 failed", Long.valueOf("10" + Integer.MAX_VALUE), NumberUtils
.createNumber("10" + Integer.MAX_VALUE + "L"));
assertEquals("createNumber(String) 17 failed", Long.valueOf("10" + Integer.MAX_VALUE), NumberUtils
.createNumber("10" + Integer.MAX_VALUE));
assertEquals("createNumber(String) 18 failed", new BigInteger("10" + Long.MAX_VALUE), NumberUtils
.createNumber("10" + Long.MAX_VALUE));
// LANG-521
assertEquals("createNumber(String) LANG-521 failed", Float.valueOf("2."), NumberUtils.createNumber("2."));
// LANG-638
assertFalse("createNumber(String) succeeded", checkCreateNumber("1eE"));
// LANG-693
assertEquals("createNumber(String) LANG-693 failed", Double.valueOf(Double.MAX_VALUE), NumberUtils
.createNumber("" + Double.MAX_VALUE));
}
@Test
public void testCreateFloat() {
assertEquals("createFloat(String) failed", Float.valueOf("1234.5"), NumberUtils.createFloat("1234.5"));
assertEquals("createFloat(null) failed", null, NumberUtils.createFloat(null));
this.testCreateFloatFailure("");
this.testCreateFloatFailure(" ");
this.testCreateFloatFailure("\b\t\n\f\r");
// Funky whitespaces
this.testCreateFloatFailure("\u00A0\uFEFF\u000B\u000C\u001C\u001D\u001E\u001F");
}
protected void testCreateFloatFailure(String str) {
try {
Float value = NumberUtils.createFloat(str);
fail("createFloat(blank) failed: " + value);
} catch (NumberFormatException ex) {
// empty
}
}
@Test
public void testCreateDouble() {
assertEquals("createDouble(String) failed", Double.valueOf("1234.5"), NumberUtils.createDouble("1234.5"));
assertEquals("createDouble(null) failed", null, NumberUtils.createDouble(null));
this.testCreateDoubleFailure("");
this.testCreateDoubleFailure(" ");
this.testCreateDoubleFailure("\b\t\n\f\r");
// Funky whitespaces
this.testCreateDoubleFailure("\u00A0\uFEFF\u000B\u000C\u001C\u001D\u001E\u001F");
}
protected void testCreateDoubleFailure(String str) {
try {
Double value = NumberUtils.createDouble(str);
fail("createDouble(blank) failed: " + value);
} catch (NumberFormatException ex) {
// empty
}
}
@Test
public void testCreateInteger() {
assertEquals("createInteger(String) failed", Integer.valueOf("12345"), NumberUtils.createInteger("12345"));
assertEquals("createInteger(null) failed", null, NumberUtils.createInteger(null));
this.testCreateIntegerFailure("");
this.testCreateIntegerFailure(" ");
this.testCreateIntegerFailure("\b\t\n\f\r");
// Funky whitespaces
this.testCreateIntegerFailure("\u00A0\uFEFF\u000B\u000C\u001C\u001D\u001E\u001F");
}
protected void testCreateIntegerFailure(String str) {
try {
Integer value = NumberUtils.createInteger(str);
fail("createInteger(blank) failed: " + value);
} catch (NumberFormatException ex) {
// empty
}
}
@Test
public void testCreateLong() {
assertEquals("createLong(String) failed", Long.valueOf("12345"), NumberUtils.createLong("12345"));
assertEquals("createLong(null) failed", null, NumberUtils.createLong(null));
this.testCreateLongFailure("");
this.testCreateLongFailure(" ");
this.testCreateLongFailure("\b\t\n\f\r");
// Funky whitespaces
this.testCreateLongFailure("\u00A0\uFEFF\u000B\u000C\u001C\u001D\u001E\u001F");
}
protected void testCreateLongFailure(String str) {
try {
Long value = NumberUtils.createLong(str);
fail("createLong(blank) failed: " + value);
} catch (NumberFormatException ex) {
// empty
}
}
@Test
public void testCreateBigInteger() {
assertEquals("createBigInteger(String) failed", new BigInteger("12345"), NumberUtils.createBigInteger("12345"));
assertEquals("createBigInteger(null) failed", null, NumberUtils.createBigInteger(null));
this.testCreateBigIntegerFailure("");
this.testCreateBigIntegerFailure(" ");
this.testCreateBigIntegerFailure("\b\t\n\f\r");
// Funky whitespaces
this.testCreateBigIntegerFailure("\u00A0\uFEFF\u000B\u000C\u001C\u001D\u001E\u001F");
}
protected void testCreateBigIntegerFailure(String str) {
try {
BigInteger value = NumberUtils.createBigInteger(str);
fail("createBigInteger(blank) failed: " + value);
} catch (NumberFormatException ex) {
// empty
}
}
@Test
public void testCreateBigDecimal() {
assertEquals("createBigDecimal(String) failed", new BigDecimal("1234.5"), NumberUtils.createBigDecimal("1234.5"));
assertEquals("createBigDecimal(null) failed", null, NumberUtils.createBigDecimal(null));
this.testCreateBigDecimalFailure("");
this.testCreateBigDecimalFailure(" ");
this.testCreateBigDecimalFailure("\b\t\n\f\r");
// Funky whitespaces
this.testCreateBigDecimalFailure("\u00A0\uFEFF\u000B\u000C\u001C\u001D\u001E\u001F");
}
protected void testCreateBigDecimalFailure(String str) {
try {
BigDecimal value = NumberUtils.createBigDecimal(str);
fail("createBigDecimal(blank) failed: " + value);
} catch (NumberFormatException ex) {
// empty
}
}
// min/max tests
// ----------------------------------------------------------------------
@Test(expected = IllegalArgumentException.class)
public void testMinLong_nullArray() {
NumberUtils.min((long[]) null);
}
@Test(expected = IllegalArgumentException.class)
public void testMinLong_emptyArray() {
NumberUtils.min(new long[0]);
}
@Test
public void testMinLong() {
assertEquals(
"min(long[]) failed for array length 1",
5,
NumberUtils.min(new long[] { 5 }));
assertEquals(
"min(long[]) failed for array length 2",
6,
NumberUtils.min(new long[] { 6, 9 }));
assertEquals(-10, NumberUtils.min(new long[] { -10, -5, 0, 5, 10 }));
assertEquals(-10, NumberUtils.min(new long[] { -5, 0, -10, 5, 10 }));
}
@Test(expected = IllegalArgumentException.class)
public void testMinInt_nullArray() {
NumberUtils.min((int[]) null);
}
@Test(expected = IllegalArgumentException.class)
public void testMinInt_emptyArray() {
NumberUtils.min(new int[0]);
}
@Test
public void testMinInt() {
assertEquals(
"min(int[]) failed for array length 1",
5,
NumberUtils.min(new int[] { 5 }));
assertEquals(
"min(int[]) failed for array length 2",
6,
NumberUtils.min(new int[] { 6, 9 }));
assertEquals(-10, NumberUtils.min(new int[] { -10, -5, 0, 5, 10 }));
assertEquals(-10, NumberUtils.min(new int[] { -5, 0, -10, 5, 10 }));
}
@Test(expected = IllegalArgumentException.class)
public void testMinShort_nullArray() {
NumberUtils.min((short[]) null);
}
@Test(expected = IllegalArgumentException.class)
public void testMinShort_emptyArray() {
NumberUtils.min(new short[0]);
}
@Test
public void testMinShort() {
assertEquals(
"min(short[]) failed for array length 1",
5,
NumberUtils.min(new short[] { 5 }));
assertEquals(
"min(short[]) failed for array length 2",
6,
NumberUtils.min(new short[] { 6, 9 }));
assertEquals(-10, NumberUtils.min(new short[] { -10, -5, 0, 5, 10 }));
assertEquals(-10, NumberUtils.min(new short[] { -5, 0, -10, 5, 10 }));
}
@Test(expected = IllegalArgumentException.class)
public void testMinByte_nullArray() {
NumberUtils.min((byte[]) null);
}
@Test(expected = IllegalArgumentException.class)
public void testMinByte_emptyArray() {
NumberUtils.min(new byte[0]);
}
@Test
public void testMinByte() {
assertEquals(
"min(byte[]) failed for array length 1",
5,
NumberUtils.min(new byte[] { 5 }));
assertEquals(
"min(byte[]) failed for array length 2",
6,
NumberUtils.min(new byte[] { 6, 9 }));
assertEquals(-10, NumberUtils.min(new byte[] { -10, -5, 0, 5, 10 }));
assertEquals(-10, NumberUtils.min(new byte[] { -5, 0, -10, 5, 10 }));
}
@Test(expected = IllegalArgumentException.class)
public void testMinDouble_nullArray() {
NumberUtils.min((double[]) null);
}
@Test(expected = IllegalArgumentException.class)
public void testMinDouble_emptyArray() {
NumberUtils.min(new double[0]);
}
@Test
public void testMinDouble() {
assertEquals(
"min(double[]) failed for array length 1",
5.12,
NumberUtils.min(new double[] { 5.12 }),
0);
assertEquals(
"min(double[]) failed for array length 2",
6.23,
NumberUtils.min(new double[] { 6.23, 9.34 }),
0);
assertEquals(
"min(double[]) failed for array length 5",
-10.45,
NumberUtils.min(new double[] { -10.45, -5.56, 0, 5.67, 10.78 }),
0);
assertEquals(-10, NumberUtils.min(new double[] { -10, -5, 0, 5, 10 }), 0.0001);
assertEquals(-10, NumberUtils.min(new double[] { -5, 0, -10, 5, 10 }), 0.0001);
}
@Test(expected = IllegalArgumentException.class)
public void testMinFloat_nullArray() {
NumberUtils.min((float[]) null);
}
@Test(expected = IllegalArgumentException.class)
public void testMinFloat_emptyArray() {
NumberUtils.min(new float[0]);
}
@Test
public void testMinFloat() {
assertEquals(
"min(float[]) failed for array length 1",
5.9f,
NumberUtils.min(new float[] { 5.9f }),
0);
assertEquals(
"min(float[]) failed for array length 2",
6.8f,
NumberUtils.min(new float[] { 6.8f, 9.7f }),
0);
assertEquals(
"min(float[]) failed for array length 5",
-10.6f,
NumberUtils.min(new float[] { -10.6f, -5.5f, 0, 5.4f, 10.3f }),
0);
assertEquals(-10, NumberUtils.min(new float[] { -10, -5, 0, 5, 10 }), 0.0001f);
assertEquals(-10, NumberUtils.min(new float[] { -5, 0, -10, 5, 10 }), 0.0001f);
}
@Test(expected = IllegalArgumentException.class)
public void testMaxLong_nullArray() {
NumberUtils.max((long[]) null);
}
@Test(expected = IllegalArgumentException.class)
public void testMaxLong_emptyArray() {
NumberUtils.max(new long[0]);
}
@Test
public void testMaxLong() {
assertEquals(
"max(long[]) failed for array length 1",
5,
NumberUtils.max(new long[] { 5 }));
assertEquals(
"max(long[]) failed for array length 2",
9,
NumberUtils.max(new long[] { 6, 9 }));
assertEquals(
"max(long[]) failed for array length 5",
10,
NumberUtils.max(new long[] { -10, -5, 0, 5, 10 }));
assertEquals(10, NumberUtils.max(new long[] { -10, -5, 0, 5, 10 }));
assertEquals(10, NumberUtils.max(new long[] { -5, 0, 10, 5, -10 }));
}
@Test(expected = IllegalArgumentException.class)
public void testMaxInt_nullArray() {
NumberUtils.max((int[]) null);
}
@Test(expected = IllegalArgumentException.class)
public void testMaxInt_emptyArray() {
NumberUtils.max(new int[0]);
}
@Test
public void testMaxInt() {
assertEquals(
"max(int[]) failed for array length 1",
5,
NumberUtils.max(new int[] { 5 }));
assertEquals(
"max(int[]) failed for array length 2",
9,
NumberUtils.max(new int[] { 6, 9 }));
assertEquals(
"max(int[]) failed for array length 5",
10,
NumberUtils.max(new int[] { -10, -5, 0, 5, 10 }));
assertEquals(10, NumberUtils.max(new int[] { -10, -5, 0, 5, 10 }));
assertEquals(10, NumberUtils.max(new int[] { -5, 0, 10, 5, -10 }));
}
@Test(expected = IllegalArgumentException.class)
public void testMaxShort_nullArray() {
NumberUtils.max((short[]) null);
}
@Test(expected = IllegalArgumentException.class)
public void testMaxShort_emptyArray() {
NumberUtils.max(new short[0]);
}
@Test
public void testMaxShort() {
assertEquals(
"max(short[]) failed for array length 1",
5,
NumberUtils.max(new short[] { 5 }));
assertEquals(
"max(short[]) failed for array length 2",
9,
NumberUtils.max(new short[] { 6, 9 }));
assertEquals(
"max(short[]) failed for array length 5",
10,
NumberUtils.max(new short[] { -10, -5, 0, 5, 10 }));
assertEquals(10, NumberUtils.max(new short[] { -10, -5, 0, 5, 10 }));
assertEquals(10, NumberUtils.max(new short[] { -5, 0, 10, 5, -10 }));
}
@Test(expected = IllegalArgumentException.class)
public void testMaxByte_nullArray() {
NumberUtils.max((byte[]) null);
}
@Test(expected = IllegalArgumentException.class)
public void testMaxByte_emptyArray() {
NumberUtils.max(new byte[0]);
}
@Test
public void testMaxByte() {
assertEquals(
"max(byte[]) failed for array length 1",
5,
NumberUtils.max(new byte[] { 5 }));
assertEquals(
"max(byte[]) failed for array length 2",
9,
NumberUtils.max(new byte[] { 6, 9 }));
assertEquals(
"max(byte[]) failed for array length 5",
10,
NumberUtils.max(new byte[] { -10, -5, 0, 5, 10 }));
assertEquals(10, NumberUtils.max(new byte[] { -10, -5, 0, 5, 10 }));
assertEquals(10, NumberUtils.max(new byte[] { -5, 0, 10, 5, -10 }));
}
@Test(expected = IllegalArgumentException.class)
public void testMaxDouble_nullArray() {
NumberUtils.max((double[]) null);
}
@Test(expected = IllegalArgumentException.class)
public void testMaxDouble_emptyArray() {
NumberUtils.max(new double[0]);
}
@Test
public void testMaxDouble() {
final double[] d = null;
try {
NumberUtils.max(d);
fail("No exception was thrown for null input.");
} catch (IllegalArgumentException ex) {}
try {
NumberUtils.max(new double[0]);
fail("No exception was thrown for empty input.");
} catch (IllegalArgumentException ex) {}
assertEquals(
"max(double[]) failed for array length 1",
5.1f,
NumberUtils.max(new double[] { 5.1f }),
0);
assertEquals(
"max(double[]) failed for array length 2",
9.2f,
NumberUtils.max(new double[] { 6.3f, 9.2f }),
0);
assertEquals(
"max(double[]) failed for float length 5",
10.4f,
NumberUtils.max(new double[] { -10.5f, -5.6f, 0, 5.7f, 10.4f }),
0);
assertEquals(10, NumberUtils.max(new double[] { -10, -5, 0, 5, 10 }), 0.0001);
assertEquals(10, NumberUtils.max(new double[] { -5, 0, 10, 5, -10 }), 0.0001);
}
@Test(expected = IllegalArgumentException.class)
public void testMaxFloat_nullArray() {
NumberUtils.max((float[]) null);
}
@Test(expected = IllegalArgumentException.class)
public void testMaxFloat_emptyArray() {
NumberUtils.max(new float[0]);
}
@Test
public void testMaxFloat() {
assertEquals(
"max(float[]) failed for array length 1",
5.1f,
NumberUtils.max(new float[] { 5.1f }),
0);
assertEquals(
"max(float[]) failed for array length 2",
9.2f,
NumberUtils.max(new float[] { 6.3f, 9.2f }),
0);
assertEquals(
"max(float[]) failed for float length 5",
10.4f,
NumberUtils.max(new float[] { -10.5f, -5.6f, 0, 5.7f, 10.4f }),
0);
assertEquals(10, NumberUtils.max(new float[] { -10, -5, 0, 5, 10 }), 0.0001f);
assertEquals(10, NumberUtils.max(new float[] { -5, 0, 10, 5, -10 }), 0.0001f);
}
@Test
public void testMinimumLong() {
assertEquals("minimum(long,long,long) 1 failed", 12345L, NumberUtils.min(12345L, 12345L + 1L, 12345L + 2L));
assertEquals("minimum(long,long,long) 2 failed", 12345L, NumberUtils.min(12345L + 1L, 12345L, 12345 + 2L));
assertEquals("minimum(long,long,long) 3 failed", 12345L, NumberUtils.min(12345L + 1L, 12345L + 2L, 12345L));
assertEquals("minimum(long,long,long) 4 failed", 12345L, NumberUtils.min(12345L + 1L, 12345L, 12345L));
assertEquals("minimum(long,long,long) 5 failed", 12345L, NumberUtils.min(12345L, 12345L, 12345L));
}
@Test
public void testMinimumInt() {
assertEquals("minimum(int,int,int) 1 failed", 12345, NumberUtils.min(12345, 12345 + 1, 12345 + 2));
assertEquals("minimum(int,int,int) 2 failed", 12345, NumberUtils.min(12345 + 1, 12345, 12345 + 2));
assertEquals("minimum(int,int,int) 3 failed", 12345, NumberUtils.min(12345 + 1, 12345 + 2, 12345));
assertEquals("minimum(int,int,int) 4 failed", 12345, NumberUtils.min(12345 + 1, 12345, 12345));
assertEquals("minimum(int,int,int) 5 failed", 12345, NumberUtils.min(12345, 12345, 12345));
}
@Test
public void testMinimumShort() {
short low = 1234;
short mid = 1234 + 1;
short high = 1234 + 2;
assertEquals("minimum(short,short,short) 1 failed", low, NumberUtils.min(low, mid, high));
assertEquals("minimum(short,short,short) 1 failed", low, NumberUtils.min(mid, low, high));
assertEquals("minimum(short,short,short) 1 failed", low, NumberUtils.min(mid, high, low));
assertEquals("minimum(short,short,short) 1 failed", low, NumberUtils.min(low, mid, low));
}
@Test
public void testMinimumByte() {
byte low = 123;
byte mid = 123 + 1;
byte high = 123 + 2;
assertEquals("minimum(byte,byte,byte) 1 failed", low, NumberUtils.min(low, mid, high));
assertEquals("minimum(byte,byte,byte) 1 failed", low, NumberUtils.min(mid, low, high));
assertEquals("minimum(byte,byte,byte) 1 failed", low, NumberUtils.min(mid, high, low));
assertEquals("minimum(byte,byte,byte) 1 failed", low, NumberUtils.min(low, mid, low));
}
@Test
public void testMinimumDouble() {
double low = 12.3;
double mid = 12.3 + 1;
double high = 12.3 + 2;
assertEquals(low, NumberUtils.min(low, mid, high), 0.0001);
assertEquals(low, NumberUtils.min(mid, low, high), 0.0001);
assertEquals(low, NumberUtils.min(mid, high, low), 0.0001);
assertEquals(low, NumberUtils.min(low, mid, low), 0.0001);
assertEquals(mid, NumberUtils.min(high, mid, high), 0.0001);
}
@Test
public void testMinimumFloat() {
float low = 12.3f;
float mid = 12.3f + 1;
float high = 12.3f + 2;
assertEquals(low, NumberUtils.min(low, mid, high), 0.0001f);
assertEquals(low, NumberUtils.min(mid, low, high), 0.0001f);
assertEquals(low, NumberUtils.min(mid, high, low), 0.0001f);
assertEquals(low, NumberUtils.min(low, mid, low), 0.0001f);
assertEquals(mid, NumberUtils.min(high, mid, high), 0.0001f);
}
@Test
public void testMaximumLong() {
assertEquals("maximum(long,long,long) 1 failed", 12345L, NumberUtils.max(12345L, 12345L - 1L, 12345L - 2L));
assertEquals("maximum(long,long,long) 2 failed", 12345L, NumberUtils.max(12345L - 1L, 12345L, 12345L - 2L));
assertEquals("maximum(long,long,long) 3 failed", 12345L, NumberUtils.max(12345L - 1L, 12345L - 2L, 12345L));
assertEquals("maximum(long,long,long) 4 failed", 12345L, NumberUtils.max(12345L - 1L, 12345L, 12345L));
assertEquals("maximum(long,long,long) 5 failed", 12345L, NumberUtils.max(12345L, 12345L, 12345L));
}
@Test
public void testMaximumInt() {
assertEquals("maximum(int,int,int) 1 failed", 12345, NumberUtils.max(12345, 12345 - 1, 12345 - 2));
assertEquals("maximum(int,int,int) 2 failed", 12345, NumberUtils.max(12345 - 1, 12345, 12345 - 2));
assertEquals("maximum(int,int,int) 3 failed", 12345, NumberUtils.max(12345 - 1, 12345 - 2, 12345));
assertEquals("maximum(int,int,int) 4 failed", 12345, NumberUtils.max(12345 - 1, 12345, 12345));
assertEquals("maximum(int,int,int) 5 failed", 12345, NumberUtils.max(12345, 12345, 12345));
}
@Test
public void testMaximumShort() {
short low = 1234;
short mid = 1234 + 1;
short high = 1234 + 2;
assertEquals("maximum(short,short,short) 1 failed", high, NumberUtils.max(low, mid, high));
assertEquals("maximum(short,short,short) 1 failed", high, NumberUtils.max(mid, low, high));
assertEquals("maximum(short,short,short) 1 failed", high, NumberUtils.max(mid, high, low));
assertEquals("maximum(short,short,short) 1 failed", high, NumberUtils.max(high, mid, high));
}
@Test
public void testMaximumByte() {
byte low = 123;
byte mid = 123 + 1;
byte high = 123 + 2;
assertEquals("maximum(byte,byte,byte) 1 failed", high, NumberUtils.max(low, mid, high));
assertEquals("maximum(byte,byte,byte) 1 failed", high, NumberUtils.max(mid, low, high));
assertEquals("maximum(byte,byte,byte) 1 failed", high, NumberUtils.max(mid, high, low));
assertEquals("maximum(byte,byte,byte) 1 failed", high, NumberUtils.max(high, mid, high));
}
@Test
public void testMaximumDouble() {
double low = 12.3;
double mid = 12.3 + 1;
double high = 12.3 + 2;
assertEquals(high, NumberUtils.max(low, mid, high), 0.0001);
assertEquals(high, NumberUtils.max(mid, low, high), 0.0001);
assertEquals(high, NumberUtils.max(mid, high, low), 0.0001);
assertEquals(mid, NumberUtils.max(low, mid, low), 0.0001);
assertEquals(high, NumberUtils.max(high, mid, high), 0.0001);
}
@Test
public void testMaximumFloat() {
float low = 12.3f;
float mid = 12.3f + 1;
float high = 12.3f + 2;
assertEquals(high, NumberUtils.max(low, mid, high), 0.0001f);
assertEquals(high, NumberUtils.max(mid, low, high), 0.0001f);
assertEquals(high, NumberUtils.max(mid, high, low), 0.0001f);
assertEquals(mid, NumberUtils.max(low, mid, low), 0.0001f);
assertEquals(high, NumberUtils.max(high, mid, high), 0.0001f);
}
// Testing JDK against old Lang functionality
@Test
public void testCompareDouble() {
assertTrue(Double.compare(Double.NaN, Double.NaN) == 0);
assertTrue(Double.compare(Double.NaN, Double.POSITIVE_INFINITY) == +1);
assertTrue(Double.compare(Double.NaN, Double.MAX_VALUE) == +1);
assertTrue(Double.compare(Double.NaN, 1.2d) == +1);
assertTrue(Double.compare(Double.NaN, 0.0d) == +1);
assertTrue(Double.compare(Double.NaN, -0.0d) == +1);
assertTrue(Double.compare(Double.NaN, -1.2d) == +1);
assertTrue(Double.compare(Double.NaN, -Double.MAX_VALUE) == +1);
assertTrue(Double.compare(Double.NaN, Double.NEGATIVE_INFINITY) == +1);
assertTrue(Double.compare(Double.POSITIVE_INFINITY, Double.NaN) == -1);
assertTrue(Double.compare(Double.POSITIVE_INFINITY, Double.POSITIVE_INFINITY) == 0);
assertTrue(Double.compare(Double.POSITIVE_INFINITY, Double.MAX_VALUE) == +1);
assertTrue(Double.compare(Double.POSITIVE_INFINITY, 1.2d) == +1);
assertTrue(Double.compare(Double.POSITIVE_INFINITY, 0.0d) == +1);
assertTrue(Double.compare(Double.POSITIVE_INFINITY, -0.0d) == +1);
assertTrue(Double.compare(Double.POSITIVE_INFINITY, -1.2d) == +1);
assertTrue(Double.compare(Double.POSITIVE_INFINITY, -Double.MAX_VALUE) == +1);
assertTrue(Double.compare(Double.POSITIVE_INFINITY, Double.NEGATIVE_INFINITY) == +1);
assertTrue(Double.compare(Double.MAX_VALUE, Double.NaN) == -1);
assertTrue(Double.compare(Double.MAX_VALUE, Double.POSITIVE_INFINITY) == -1);
assertTrue(Double.compare(Double.MAX_VALUE, Double.MAX_VALUE) == 0);
assertTrue(Double.compare(Double.MAX_VALUE, 1.2d) == +1);
assertTrue(Double.compare(Double.MAX_VALUE, 0.0d) == +1);
assertTrue(Double.compare(Double.MAX_VALUE, -0.0d) == +1);
assertTrue(Double.compare(Double.MAX_VALUE, -1.2d) == +1);
assertTrue(Double.compare(Double.MAX_VALUE, -Double.MAX_VALUE) == +1);
assertTrue(Double.compare(Double.MAX_VALUE, Double.NEGATIVE_INFINITY) == +1);
assertTrue(Double.compare(1.2d, Double.NaN) == -1);
assertTrue(Double.compare(1.2d, Double.POSITIVE_INFINITY) == -1);
assertTrue(Double.compare(1.2d, Double.MAX_VALUE) == -1);
assertTrue(Double.compare(1.2d, 1.2d) == 0);
assertTrue(Double.compare(1.2d, 0.0d) == +1);
assertTrue(Double.compare(1.2d, -0.0d) == +1);
assertTrue(Double.compare(1.2d, -1.2d) == +1);
assertTrue(Double.compare(1.2d, -Double.MAX_VALUE) == +1);
assertTrue(Double.compare(1.2d, Double.NEGATIVE_INFINITY) == +1);
assertTrue(Double.compare(0.0d, Double.NaN) == -1);
assertTrue(Double.compare(0.0d, Double.POSITIVE_INFINITY) == -1);
assertTrue(Double.compare(0.0d, Double.MAX_VALUE) == -1);
assertTrue(Double.compare(0.0d, 1.2d) == -1);
assertTrue(Double.compare(0.0d, 0.0d) == 0);
assertTrue(Double.compare(0.0d, -0.0d) == +1);
assertTrue(Double.compare(0.0d, -1.2d) == +1);
assertTrue(Double.compare(0.0d, -Double.MAX_VALUE) == +1);
assertTrue(Double.compare(0.0d, Double.NEGATIVE_INFINITY) == +1);
assertTrue(Double.compare(-0.0d, Double.NaN) == -1);
assertTrue(Double.compare(-0.0d, Double.POSITIVE_INFINITY) == -1);
assertTrue(Double.compare(-0.0d, Double.MAX_VALUE) == -1);
assertTrue(Double.compare(-0.0d, 1.2d) == -1);
assertTrue(Double.compare(-0.0d, 0.0d) == -1);
assertTrue(Double.compare(-0.0d, -0.0d) == 0);
assertTrue(Double.compare(-0.0d, -1.2d) == +1);
assertTrue(Double.compare(-0.0d, -Double.MAX_VALUE) == +1);
assertTrue(Double.compare(-0.0d, Double.NEGATIVE_INFINITY) == +1);
assertTrue(Double.compare(-1.2d, Double.NaN) == -1);
assertTrue(Double.compare(-1.2d, Double.POSITIVE_INFINITY) == -1);
assertTrue(Double.compare(-1.2d, Double.MAX_VALUE) == -1);
assertTrue(Double.compare(-1.2d, 1.2d) == -1);
assertTrue(Double.compare(-1.2d, 0.0d) == -1);
assertTrue(Double.compare(-1.2d, -0.0d) == -1);
assertTrue(Double.compare(-1.2d, -1.2d) == 0);
assertTrue(Double.compare(-1.2d, -Double.MAX_VALUE) == +1);
assertTrue(Double.compare(-1.2d, Double.NEGATIVE_INFINITY) == +1);
assertTrue(Double.compare(-Double.MAX_VALUE, Double.NaN) == -1);
assertTrue(Double.compare(-Double.MAX_VALUE, Double.POSITIVE_INFINITY) == -1);
assertTrue(Double.compare(-Double.MAX_VALUE, Double.MAX_VALUE) == -1);
assertTrue(Double.compare(-Double.MAX_VALUE, 1.2d) == -1);
assertTrue(Double.compare(-Double.MAX_VALUE, 0.0d) == -1);
assertTrue(Double.compare(-Double.MAX_VALUE, -0.0d) == -1);
assertTrue(Double.compare(-Double.MAX_VALUE, -1.2d) == -1);
assertTrue(Double.compare(-Double.MAX_VALUE, -Double.MAX_VALUE) == 0);
assertTrue(Double.compare(-Double.MAX_VALUE, Double.NEGATIVE_INFINITY) == +1);
assertTrue(Double.compare(Double.NEGATIVE_INFINITY, Double.NaN) == -1);
assertTrue(Double.compare(Double.NEGATIVE_INFINITY, Double.POSITIVE_INFINITY) == -1);
assertTrue(Double.compare(Double.NEGATIVE_INFINITY, Double.MAX_VALUE) == -1);
assertTrue(Double.compare(Double.NEGATIVE_INFINITY, 1.2d) == -1);
assertTrue(Double.compare(Double.NEGATIVE_INFINITY, 0.0d) == -1);
assertTrue(Double.compare(Double.NEGATIVE_INFINITY, -0.0d) == -1);
assertTrue(Double.compare(Double.NEGATIVE_INFINITY, -1.2d) == -1);
assertTrue(Double.compare(Double.NEGATIVE_INFINITY, -Double.MAX_VALUE) == -1);
assertTrue(Double.compare(Double.NEGATIVE_INFINITY, Double.NEGATIVE_INFINITY) == 0);
}
@Test
public void testCompareFloat() {
assertTrue(Float.compare(Float.NaN, Float.NaN) == 0);
assertTrue(Float.compare(Float.NaN, Float.POSITIVE_INFINITY) == +1);
assertTrue(Float.compare(Float.NaN, Float.MAX_VALUE) == +1);
assertTrue(Float.compare(Float.NaN, 1.2f) == +1);
assertTrue(Float.compare(Float.NaN, 0.0f) == +1);
assertTrue(Float.compare(Float.NaN, -0.0f) == +1);
assertTrue(Float.compare(Float.NaN, -1.2f) == +1);
assertTrue(Float.compare(Float.NaN, -Float.MAX_VALUE) == +1);
assertTrue(Float.compare(Float.NaN, Float.NEGATIVE_INFINITY) == +1);
assertTrue(Float.compare(Float.POSITIVE_INFINITY, Float.NaN) == -1);
assertTrue(Float.compare(Float.POSITIVE_INFINITY, Float.POSITIVE_INFINITY) == 0);
assertTrue(Float.compare(Float.POSITIVE_INFINITY, Float.MAX_VALUE) == +1);
assertTrue(Float.compare(Float.POSITIVE_INFINITY, 1.2f) == +1);
assertTrue(Float.compare(Float.POSITIVE_INFINITY, 0.0f) == +1);
assertTrue(Float.compare(Float.POSITIVE_INFINITY, -0.0f) == +1);
assertTrue(Float.compare(Float.POSITIVE_INFINITY, -1.2f) == +1);
assertTrue(Float.compare(Float.POSITIVE_INFINITY, -Float.MAX_VALUE) == +1);
assertTrue(Float.compare(Float.POSITIVE_INFINITY, Float.NEGATIVE_INFINITY) == +1);
assertTrue(Float.compare(Float.MAX_VALUE, Float.NaN) == -1);
assertTrue(Float.compare(Float.MAX_VALUE, Float.POSITIVE_INFINITY) == -1);
assertTrue(Float.compare(Float.MAX_VALUE, Float.MAX_VALUE) == 0);
assertTrue(Float.compare(Float.MAX_VALUE, 1.2f) == +1);
assertTrue(Float.compare(Float.MAX_VALUE, 0.0f) == +1);
assertTrue(Float.compare(Float.MAX_VALUE, -0.0f) == +1);
assertTrue(Float.compare(Float.MAX_VALUE, -1.2f) == +1);
assertTrue(Float.compare(Float.MAX_VALUE, -Float.MAX_VALUE) == +1);
assertTrue(Float.compare(Float.MAX_VALUE, Float.NEGATIVE_INFINITY) == +1);
assertTrue(Float.compare(1.2f, Float.NaN) == -1);
assertTrue(Float.compare(1.2f, Float.POSITIVE_INFINITY) == -1);
assertTrue(Float.compare(1.2f, Float.MAX_VALUE) == -1);
assertTrue(Float.compare(1.2f, 1.2f) == 0);
assertTrue(Float.compare(1.2f, 0.0f) == +1);
assertTrue(Float.compare(1.2f, -0.0f) == +1);
assertTrue(Float.compare(1.2f, -1.2f) == +1);
assertTrue(Float.compare(1.2f, -Float.MAX_VALUE) == +1);
assertTrue(Float.compare(1.2f, Float.NEGATIVE_INFINITY) == +1);
assertTrue(Float.compare(0.0f, Float.NaN) == -1);
assertTrue(Float.compare(0.0f, Float.POSITIVE_INFINITY) == -1);
assertTrue(Float.compare(0.0f, Float.MAX_VALUE) == -1);
assertTrue(Float.compare(0.0f, 1.2f) == -1);
assertTrue(Float.compare(0.0f, 0.0f) == 0);
assertTrue(Float.compare(0.0f, -0.0f) == +1);
assertTrue(Float.compare(0.0f, -1.2f) == +1);
assertTrue(Float.compare(0.0f, -Float.MAX_VALUE) == +1);
assertTrue(Float.compare(0.0f, Float.NEGATIVE_INFINITY) == +1);
assertTrue(Float.compare(-0.0f, Float.NaN) == -1);
assertTrue(Float.compare(-0.0f, Float.POSITIVE_INFINITY) == -1);
assertTrue(Float.compare(-0.0f, Float.MAX_VALUE) == -1);
assertTrue(Float.compare(-0.0f, 1.2f) == -1);
assertTrue(Float.compare(-0.0f, 0.0f) == -1);
assertTrue(Float.compare(-0.0f, -0.0f) == 0);
assertTrue(Float.compare(-0.0f, -1.2f) == +1);
assertTrue(Float.compare(-0.0f, -Float.MAX_VALUE) == +1);
assertTrue(Float.compare(-0.0f, Float.NEGATIVE_INFINITY) == +1);
assertTrue(Float.compare(-1.2f, Float.NaN) == -1);
assertTrue(Float.compare(-1.2f, Float.POSITIVE_INFINITY) == -1);
assertTrue(Float.compare(-1.2f, Float.MAX_VALUE) == -1);
assertTrue(Float.compare(-1.2f, 1.2f) == -1);
assertTrue(Float.compare(-1.2f, 0.0f) == -1);
assertTrue(Float.compare(-1.2f, -0.0f) == -1);
assertTrue(Float.compare(-1.2f, -1.2f) == 0);
assertTrue(Float.compare(-1.2f, -Float.MAX_VALUE) == +1);
assertTrue(Float.compare(-1.2f, Float.NEGATIVE_INFINITY) == +1);
assertTrue(Float.compare(-Float.MAX_VALUE, Float.NaN) == -1);
assertTrue(Float.compare(-Float.MAX_VALUE, Float.POSITIVE_INFINITY) == -1);
assertTrue(Float.compare(-Float.MAX_VALUE, Float.MAX_VALUE) == -1);
assertTrue(Float.compare(-Float.MAX_VALUE, 1.2f) == -1);
assertTrue(Float.compare(-Float.MAX_VALUE, 0.0f) == -1);
assertTrue(Float.compare(-Float.MAX_VALUE, -0.0f) == -1);
assertTrue(Float.compare(-Float.MAX_VALUE, -1.2f) == -1);
assertTrue(Float.compare(-Float.MAX_VALUE, -Float.MAX_VALUE) == 0);
assertTrue(Float.compare(-Float.MAX_VALUE, Float.NEGATIVE_INFINITY) == +1);
assertTrue(Float.compare(Float.NEGATIVE_INFINITY, Float.NaN) == -1);
assertTrue(Float.compare(Float.NEGATIVE_INFINITY, Float.POSITIVE_INFINITY) == -1);
assertTrue(Float.compare(Float.NEGATIVE_INFINITY, Float.MAX_VALUE) == -1);
assertTrue(Float.compare(Float.NEGATIVE_INFINITY, 1.2f) == -1);
assertTrue(Float.compare(Float.NEGATIVE_INFINITY, 0.0f) == -1);
assertTrue(Float.compare(Float.NEGATIVE_INFINITY, -0.0f) == -1);
assertTrue(Float.compare(Float.NEGATIVE_INFINITY, -1.2f) == -1);
assertTrue(Float.compare(Float.NEGATIVE_INFINITY, -Float.MAX_VALUE) == -1);
assertTrue(Float.compare(Float.NEGATIVE_INFINITY, Float.NEGATIVE_INFINITY) == 0);
}
@Test
public void testIsDigits() {
assertFalse("isDigits(null) failed", NumberUtils.isDigits(null));
assertFalse("isDigits('') failed", NumberUtils.isDigits(""));
assertTrue("isDigits(String) failed", NumberUtils.isDigits("12345"));
assertFalse("isDigits(String) neg 1 failed", NumberUtils.isDigits("1234.5"));
assertFalse("isDigits(String) neg 3 failed", NumberUtils.isDigits("1ab"));
assertFalse("isDigits(String) neg 4 failed", NumberUtils.isDigits("abc"));
}
/**
* Tests isNumber(String) and tests that createNumber(String) returns
* a valid number iff isNumber(String) returns false.
*/
@Test
public void testIsNumber() {
String val = "12345";
assertTrue("isNumber(String) 1 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 1 failed", checkCreateNumber(val));
val = "1234.5";
assertTrue("isNumber(String) 2 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 2 failed", checkCreateNumber(val));
val = ".12345";
assertTrue("isNumber(String) 3 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 3 failed", checkCreateNumber(val));
val = "1234E5";
assertTrue("isNumber(String) 4 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 4 failed", checkCreateNumber(val));
val = "1234E+5";
assertTrue("isNumber(String) 5 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 5 failed", checkCreateNumber(val));
val = "1234E-5";
assertTrue("isNumber(String) 6 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 6 failed", checkCreateNumber(val));
val = "123.4E5";
assertTrue("isNumber(String) 7 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 7 failed", checkCreateNumber(val));
val = "-1234";
assertTrue("isNumber(String) 8 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 8 failed", checkCreateNumber(val));
val = "-1234.5";
assertTrue("isNumber(String) 9 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 9 failed", checkCreateNumber(val));
val = "-.12345";
assertTrue("isNumber(String) 10 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 10 failed", checkCreateNumber(val));
val = "-1234E5";
assertTrue("isNumber(String) 11 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 11 failed", checkCreateNumber(val));
val = "0";
assertTrue("isNumber(String) 12 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 12 failed", checkCreateNumber(val));
val = "-0";
assertTrue("isNumber(String) 13 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 13 failed", checkCreateNumber(val));
val = "01234";
assertTrue("isNumber(String) 14 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 14 failed", checkCreateNumber(val));
val = "-01234";
assertTrue("isNumber(String) 15 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 15 failed", checkCreateNumber(val));
val = "0xABC123";
assertTrue("isNumber(String) 16 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 16 failed", checkCreateNumber(val));
val = "0x0";
assertTrue("isNumber(String) 17 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 17 failed", checkCreateNumber(val));
val = "123.4E21D";
assertTrue("isNumber(String) 19 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 19 failed", checkCreateNumber(val));
val = "-221.23F";
assertTrue("isNumber(String) 20 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 20 failed", checkCreateNumber(val));
val = "22338L";
assertTrue("isNumber(String) 21 failed", NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 21 failed", checkCreateNumber(val));
val = null;
assertTrue("isNumber(String) 1 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 1 Neg failed", !checkCreateNumber(val));
val = "";
assertTrue("isNumber(String) 2 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 2 Neg failed", !checkCreateNumber(val));
val = "--2.3";
assertTrue("isNumber(String) 3 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 3 Neg failed", !checkCreateNumber(val));
val = ".12.3";
assertTrue("isNumber(String) 4 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 4 Neg failed", !checkCreateNumber(val));
val = "-123E";
assertTrue("isNumber(String) 5 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 5 Neg failed", !checkCreateNumber(val));
val = "-123E+-212";
assertTrue("isNumber(String) 6 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 6 Neg failed", !checkCreateNumber(val));
val = "-123E2.12";
assertTrue("isNumber(String) 7 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 7 Neg failed", !checkCreateNumber(val));
val = "0xGF";
assertTrue("isNumber(String) 8 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 8 Neg failed", !checkCreateNumber(val));
val = "0xFAE-1";
assertTrue("isNumber(String) 9 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 9 Neg failed", !checkCreateNumber(val));
val = ".";
assertTrue("isNumber(String) 10 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 10 Neg failed", !checkCreateNumber(val));
val = "-0ABC123";
assertTrue("isNumber(String) 11 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 11 Neg failed", !checkCreateNumber(val));
val = "123.4E-D";
assertTrue("isNumber(String) 12 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 12 Neg failed", !checkCreateNumber(val));
val = "123.4ED";
assertTrue("isNumber(String) 13 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 13 Neg failed", !checkCreateNumber(val));
val = "1234E5l";
assertTrue("isNumber(String) 14 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 14 Neg failed", !checkCreateNumber(val));
val = "11a";
assertTrue("isNumber(String) 15 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 15 Neg failed", !checkCreateNumber(val));
val = "1a";
assertTrue("isNumber(String) 16 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 16 Neg failed", !checkCreateNumber(val));
val = "a";
assertTrue("isNumber(String) 17 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 17 Neg failed", !checkCreateNumber(val));
val = "11g";
assertTrue("isNumber(String) 18 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 18 Neg failed", !checkCreateNumber(val));
val = "11z";
assertTrue("isNumber(String) 19 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 19 Neg failed", !checkCreateNumber(val));
val = "11def";
assertTrue("isNumber(String) 20 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 20 Neg failed", !checkCreateNumber(val));
val = "11d11";
assertTrue("isNumber(String) 21 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 21 Neg failed", !checkCreateNumber(val));
val = "11 11";
assertTrue("isNumber(String) 22 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 22 Neg failed", !checkCreateNumber(val));
val = " 1111";
assertTrue("isNumber(String) 23 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 23 Neg failed", !checkCreateNumber(val));
val = "1111 ";
assertTrue("isNumber(String) 24 Neg failed", !NumberUtils.isNumber(val));
assertTrue("isNumber(String)/createNumber(String) 24 Neg failed", !checkCreateNumber(val));
// LANG-521
val = "2.";
assertTrue("isNumber(String) LANG-521 failed", NumberUtils.isNumber(val));
// LANG-664
val = "1.1L";
assertFalse("isNumber(String) LANG-664 failed", NumberUtils.isNumber(val));
}
private boolean checkCreateNumber(String val) {
try {
Object obj = NumberUtils.createNumber(val);
if (obj == null) {
return false;
}
return true;
} catch (NumberFormatException e) {
return false;
}
}
@SuppressWarnings("cast") // suppress instanceof warning check
@Test
public void testConstants() {
assertTrue(NumberUtils.LONG_ZERO instanceof Long);
assertTrue(NumberUtils.LONG_ONE instanceof Long);
assertTrue(NumberUtils.LONG_MINUS_ONE instanceof Long);
assertTrue(NumberUtils.INTEGER_ZERO instanceof Integer);
assertTrue(NumberUtils.INTEGER_ONE instanceof Integer);
assertTrue(NumberUtils.INTEGER_MINUS_ONE instanceof Integer);
assertTrue(NumberUtils.SHORT_ZERO instanceof Short);
assertTrue(NumberUtils.SHORT_ONE instanceof Short);
assertTrue(NumberUtils.SHORT_MINUS_ONE instanceof Short);
assertTrue(NumberUtils.BYTE_ZERO instanceof Byte);
assertTrue(NumberUtils.BYTE_ONE instanceof Byte);
assertTrue(NumberUtils.BYTE_MINUS_ONE instanceof Byte);
assertTrue(NumberUtils.DOUBLE_ZERO instanceof Double);
assertTrue(NumberUtils.DOUBLE_ONE instanceof Double);
assertTrue(NumberUtils.DOUBLE_MINUS_ONE instanceof Double);
assertTrue(NumberUtils.FLOAT_ZERO instanceof Float);
assertTrue(NumberUtils.FLOAT_ONE instanceof Float);
assertTrue(NumberUtils.FLOAT_MINUS_ONE instanceof Float);
assertTrue(NumberUtils.LONG_ZERO.longValue() == 0);
assertTrue(NumberUtils.LONG_ONE.longValue() == 1);
assertTrue(NumberUtils.LONG_MINUS_ONE.longValue() == -1);
assertTrue(NumberUtils.INTEGER_ZERO.intValue() == 0);
assertTrue(NumberUtils.INTEGER_ONE.intValue() == 1);
assertTrue(NumberUtils.INTEGER_MINUS_ONE.intValue() == -1);
assertTrue(NumberUtils.SHORT_ZERO.shortValue() == 0);
assertTrue(NumberUtils.SHORT_ONE.shortValue() == 1);
assertTrue(NumberUtils.SHORT_MINUS_ONE.shortValue() == -1);
assertTrue(NumberUtils.BYTE_ZERO.byteValue() == 0);
assertTrue(NumberUtils.BYTE_ONE.byteValue() == 1);
assertTrue(NumberUtils.BYTE_MINUS_ONE.byteValue() == -1);
assertTrue(NumberUtils.DOUBLE_ZERO.doubleValue() == 0.0d);
assertTrue(NumberUtils.DOUBLE_ONE.doubleValue() == 1.0d);
assertTrue(NumberUtils.DOUBLE_MINUS_ONE.doubleValue() == -1.0d);
assertTrue(NumberUtils.FLOAT_ZERO.floatValue() == 0.0f);
assertTrue(NumberUtils.FLOAT_ONE.floatValue() == 1.0f);
assertTrue(NumberUtils.FLOAT_MINUS_ONE.floatValue() == -1.0f);
}
@Test
public void testLang300() {
NumberUtils.createNumber("-1l");
NumberUtils.createNumber("01l");
NumberUtils.createNumber("1l");
}
@Test
public void testLang381() {
assertTrue(Double.isNaN(NumberUtils.min(1.2, 2.5, Double.NaN)));
assertTrue(Double.isNaN(NumberUtils.max(1.2, 2.5, Double.NaN)));
assertTrue(Float.isNaN(NumberUtils.min(1.2f, 2.5f, Float.NaN)));
assertTrue(Float.isNaN(NumberUtils.max(1.2f, 2.5f, Float.NaN)));
double[] a = new double[] { 1.2, Double.NaN, 3.7, 27.0, 42.0, Double.NaN };
assertTrue(Double.isNaN(NumberUtils.max(a)));
assertTrue(Double.isNaN(NumberUtils.min(a)));
double[] b = new double[] { Double.NaN, 1.2, Double.NaN, 3.7, 27.0, 42.0, Double.NaN };
assertTrue(Double.isNaN(NumberUtils.max(b)));
assertTrue(Double.isNaN(NumberUtils.min(b)));
float[] aF = new float[] { 1.2f, Float.NaN, 3.7f, 27.0f, 42.0f, Float.NaN };
assertTrue(Float.isNaN(NumberUtils.max(aF)));
float[] bF = new float[] { Float.NaN, 1.2f, Float.NaN, 3.7f, 27.0f, 42.0f, Float.NaN };
assertTrue(Float.isNaN(NumberUtils.max(bF)));
}
} |
||
public void testRecursiveRecord() {
ProxyObjectType loop = new ProxyObjectType(registry, NUMBER_TYPE);
JSType record = new RecordTypeBuilder(registry)
.addProperty("loop", loop, null)
.addProperty("number", NUMBER_TYPE, null)
.addProperty("string", STRING_TYPE, null)
.build();
assertEquals("{loop: number, number: number, string: string}",
record.toString());
loop.setReferencedType(record);
assertEquals("{loop: {...}, number: number, string: string}",
record.toString());
assertEquals("{loop: ?, number: number, string: string}",
record.toAnnotationString());
Asserts.assertEquivalenceOperations(record, loop);
} | com.google.javascript.rhino.jstype.RecordTypeTest::testRecursiveRecord | test/com/google/javascript/rhino/jstype/RecordTypeTest.java | 62 | test/com/google/javascript/rhino/jstype/RecordTypeTest.java | testRecursiveRecord | /*
*
* ***** BEGIN LICENSE BLOCK *****
* Version: MPL 1.1/GPL 2.0
*
* The contents of this file are subject to the Mozilla Public License Version
* 1.1 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
* http://www.mozilla.org/MPL/
*
* Software distributed under the License is distributed on an "AS IS" basis,
* WITHOUT WARRANTY OF ANY KIND, either express or implied. See the License
* for the specific language governing rights and limitations under the
* License.
*
* The Original Code is Rhino code, released
* May 6, 1999.
*
* The Initial Developer of the Original Code is
* Netscape Communications Corporation.
* Portions created by the Initial Developer are Copyright (C) 1997-1999
* the Initial Developer. All Rights Reserved.
*
* Contributor(s):
* Nick Santos
*
* Alternatively, the contents of this file may be used under the terms of
* the GNU General Public License Version 2 or later (the "GPL"), in which
* case the provisions of the GPL are applicable instead of those above. If
* you wish to allow use of your version of this file only under the terms of
* the GPL and not to allow others to use your version of this file under the
* MPL, indicate your decision by deleting the provisions above and replacing
* them with the notice and other provisions required by the GPL. If you do
* not delete the provisions above, a recipient may use your version of this
* file under either the MPL or the GPL.
*
* ***** END LICENSE BLOCK ***** */
package com.google.javascript.rhino.jstype;
import com.google.javascript.rhino.testing.Asserts;
import com.google.javascript.rhino.testing.BaseJSTypeTestCase;
public class RecordTypeTest extends BaseJSTypeTestCase {
public void testRecursiveRecord() {
ProxyObjectType loop = new ProxyObjectType(registry, NUMBER_TYPE);
JSType record = new RecordTypeBuilder(registry)
.addProperty("loop", loop, null)
.addProperty("number", NUMBER_TYPE, null)
.addProperty("string", STRING_TYPE, null)
.build();
assertEquals("{loop: number, number: number, string: string}",
record.toString());
loop.setReferencedType(record);
assertEquals("{loop: {...}, number: number, string: string}",
record.toString());
assertEquals("{loop: ?, number: number, string: string}",
record.toAnnotationString());
Asserts.assertEquivalenceOperations(record, loop);
}
public void testLongToString() {
JSType record = new RecordTypeBuilder(registry)
.addProperty("a1", NUMBER_TYPE, null)
.addProperty("a2", NUMBER_TYPE, null)
.addProperty("a3", NUMBER_TYPE, null)
.addProperty("a4", NUMBER_TYPE, null)
.addProperty("a5", NUMBER_TYPE, null)
.addProperty("a6", NUMBER_TYPE, null)
.build();
assertEquals("{a1: number, a2: number, a3: number, a4: number, ...}",
record.toString());
assertEquals(
"{a1: number, a2: number, a3: number, a4: number," +
" a5: number, a6: number}",
record.toAnnotationString());
}
public void testSupAndInf() {
JSType recordA = new RecordTypeBuilder(registry)
.addProperty("a", NUMBER_TYPE, null)
.addProperty("b", NUMBER_TYPE, null)
.build();
JSType recordC = new RecordTypeBuilder(registry)
.addProperty("b", NUMBER_TYPE, null)
.addProperty("c", NUMBER_TYPE, null)
.build();
ProxyObjectType proxyRecordA = new ProxyObjectType(registry, recordA);
ProxyObjectType proxyRecordC = new ProxyObjectType(registry, recordC);
JSType aInfC = new RecordTypeBuilder(registry)
.addProperty("a", NUMBER_TYPE, null)
.addProperty("b", NUMBER_TYPE, null)
.addProperty("c", NUMBER_TYPE, null)
.build();
JSType aSupC = registry.createUnionType(recordA, recordC);
Asserts.assertTypeEquals(
aInfC, recordA.getGreatestSubtype(recordC));
Asserts.assertTypeEquals(
aSupC, recordA.getLeastSupertype(recordC));
Asserts.assertTypeEquals(
aInfC, proxyRecordA.getGreatestSubtype(proxyRecordC));
Asserts.assertTypeEquals(
aSupC, proxyRecordA.getLeastSupertype(proxyRecordC));
}
} | // You are a professional Java test case writer, please create a test case named `testRecursiveRecord` for the issue `Closure-643`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-643
//
// ## Issue-Title:
// externExport with @typedef can generate invalid externs
//
// ## Issue-Description:
// **What steps will reproduce the problem?**
// 1. Create a file that has a @typedef and code referencing the type def above and below the typedef declaration.
// 2. Run the closure compiler and grab the externExport string stored on the last result for review.
// 3. I have attached both source and output files displaying the issue.
//
// **What is the expected output? What do you see instead?**
//
// The code above the @typedef references the aliased name of the @typedef as expected however the code below the @typedef tries embedding the body of the @typedef and ends up truncating it if the length is too long with a "...". This throws bad type errors when compiling against this extern. What is odd is this only seems to be the case when the parameter with the type is optional. When neither are optional it embeds the types, which is not a big deal, except when types are long; they get truncated and throw errors.
//
//
// **What version of the product are you using? On what operating system?**
//
// plovr built from revision 3103:d6db24beeb7f
// Revision numbers for embedded Closure Tools:
// Closure Library: 1374
// Closure Compiler: 1559
// Closure Templates: 23
//
// **Please provide any additional information below.**
//
//
public void testRecursiveRecord() {
| 62 | 39 | 45 | test/com/google/javascript/rhino/jstype/RecordTypeTest.java | test | ```markdown
## Issue-ID: Closure-643
## Issue-Title:
externExport with @typedef can generate invalid externs
## Issue-Description:
**What steps will reproduce the problem?**
1. Create a file that has a @typedef and code referencing the type def above and below the typedef declaration.
2. Run the closure compiler and grab the externExport string stored on the last result for review.
3. I have attached both source and output files displaying the issue.
**What is the expected output? What do you see instead?**
The code above the @typedef references the aliased name of the @typedef as expected however the code below the @typedef tries embedding the body of the @typedef and ends up truncating it if the length is too long with a "...". This throws bad type errors when compiling against this extern. What is odd is this only seems to be the case when the parameter with the type is optional. When neither are optional it embeds the types, which is not a big deal, except when types are long; they get truncated and throw errors.
**What version of the product are you using? On what operating system?**
plovr built from revision 3103:d6db24beeb7f
Revision numbers for embedded Closure Tools:
Closure Library: 1374
Closure Compiler: 1559
Closure Templates: 23
**Please provide any additional information below.**
```
You are a professional Java test case writer, please create a test case named `testRecursiveRecord` for the issue `Closure-643`, utilizing the provided issue report information and the following function signature.
```java
public void testRecursiveRecord() {
```
| 45 | [
"com.google.javascript.rhino.jstype.PrototypeObjectType"
] | 8975a77b5651a4e38748eaeb5f5b4ce0e0b56d6ba507ce44b1cae34cb3d5766c | public void testRecursiveRecord() | // You are a professional Java test case writer, please create a test case named `testRecursiveRecord` for the issue `Closure-643`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-643
//
// ## Issue-Title:
// externExport with @typedef can generate invalid externs
//
// ## Issue-Description:
// **What steps will reproduce the problem?**
// 1. Create a file that has a @typedef and code referencing the type def above and below the typedef declaration.
// 2. Run the closure compiler and grab the externExport string stored on the last result for review.
// 3. I have attached both source and output files displaying the issue.
//
// **What is the expected output? What do you see instead?**
//
// The code above the @typedef references the aliased name of the @typedef as expected however the code below the @typedef tries embedding the body of the @typedef and ends up truncating it if the length is too long with a "...". This throws bad type errors when compiling against this extern. What is odd is this only seems to be the case when the parameter with the type is optional. When neither are optional it embeds the types, which is not a big deal, except when types are long; they get truncated and throw errors.
//
//
// **What version of the product are you using? On what operating system?**
//
// plovr built from revision 3103:d6db24beeb7f
// Revision numbers for embedded Closure Tools:
// Closure Library: 1374
// Closure Compiler: 1559
// Closure Templates: 23
//
// **Please provide any additional information below.**
//
//
| Closure | /*
*
* ***** BEGIN LICENSE BLOCK *****
* Version: MPL 1.1/GPL 2.0
*
* The contents of this file are subject to the Mozilla Public License Version
* 1.1 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
* http://www.mozilla.org/MPL/
*
* Software distributed under the License is distributed on an "AS IS" basis,
* WITHOUT WARRANTY OF ANY KIND, either express or implied. See the License
* for the specific language governing rights and limitations under the
* License.
*
* The Original Code is Rhino code, released
* May 6, 1999.
*
* The Initial Developer of the Original Code is
* Netscape Communications Corporation.
* Portions created by the Initial Developer are Copyright (C) 1997-1999
* the Initial Developer. All Rights Reserved.
*
* Contributor(s):
* Nick Santos
*
* Alternatively, the contents of this file may be used under the terms of
* the GNU General Public License Version 2 or later (the "GPL"), in which
* case the provisions of the GPL are applicable instead of those above. If
* you wish to allow use of your version of this file only under the terms of
* the GPL and not to allow others to use your version of this file under the
* MPL, indicate your decision by deleting the provisions above and replacing
* them with the notice and other provisions required by the GPL. If you do
* not delete the provisions above, a recipient may use your version of this
* file under either the MPL or the GPL.
*
* ***** END LICENSE BLOCK ***** */
package com.google.javascript.rhino.jstype;
import com.google.javascript.rhino.testing.Asserts;
import com.google.javascript.rhino.testing.BaseJSTypeTestCase;
public class RecordTypeTest extends BaseJSTypeTestCase {
public void testRecursiveRecord() {
ProxyObjectType loop = new ProxyObjectType(registry, NUMBER_TYPE);
JSType record = new RecordTypeBuilder(registry)
.addProperty("loop", loop, null)
.addProperty("number", NUMBER_TYPE, null)
.addProperty("string", STRING_TYPE, null)
.build();
assertEquals("{loop: number, number: number, string: string}",
record.toString());
loop.setReferencedType(record);
assertEquals("{loop: {...}, number: number, string: string}",
record.toString());
assertEquals("{loop: ?, number: number, string: string}",
record.toAnnotationString());
Asserts.assertEquivalenceOperations(record, loop);
}
public void testLongToString() {
JSType record = new RecordTypeBuilder(registry)
.addProperty("a1", NUMBER_TYPE, null)
.addProperty("a2", NUMBER_TYPE, null)
.addProperty("a3", NUMBER_TYPE, null)
.addProperty("a4", NUMBER_TYPE, null)
.addProperty("a5", NUMBER_TYPE, null)
.addProperty("a6", NUMBER_TYPE, null)
.build();
assertEquals("{a1: number, a2: number, a3: number, a4: number, ...}",
record.toString());
assertEquals(
"{a1: number, a2: number, a3: number, a4: number," +
" a5: number, a6: number}",
record.toAnnotationString());
}
public void testSupAndInf() {
JSType recordA = new RecordTypeBuilder(registry)
.addProperty("a", NUMBER_TYPE, null)
.addProperty("b", NUMBER_TYPE, null)
.build();
JSType recordC = new RecordTypeBuilder(registry)
.addProperty("b", NUMBER_TYPE, null)
.addProperty("c", NUMBER_TYPE, null)
.build();
ProxyObjectType proxyRecordA = new ProxyObjectType(registry, recordA);
ProxyObjectType proxyRecordC = new ProxyObjectType(registry, recordC);
JSType aInfC = new RecordTypeBuilder(registry)
.addProperty("a", NUMBER_TYPE, null)
.addProperty("b", NUMBER_TYPE, null)
.addProperty("c", NUMBER_TYPE, null)
.build();
JSType aSupC = registry.createUnionType(recordA, recordC);
Asserts.assertTypeEquals(
aInfC, recordA.getGreatestSubtype(recordC));
Asserts.assertTypeEquals(
aSupC, recordA.getLeastSupertype(recordC));
Asserts.assertTypeEquals(
aInfC, proxyRecordA.getGreatestSubtype(proxyRecordC));
Asserts.assertTypeEquals(
aSupC, proxyRecordA.getLeastSupertype(proxyRecordC));
}
} |
||
public void testLocalType728() throws Exception
{
TypeFactory tf = TypeFactory.defaultInstance();
Method m = Issue728.class.getMethod("method", CharSequence.class);
assertNotNull(m);
// Start with return type
// first type-erased
JavaType t = tf.constructType(m.getReturnType());
assertEquals(CharSequence.class, t.getRawClass());
// then generic
t = tf.constructType(m.getGenericReturnType());
assertEquals(CharSequence.class, t.getRawClass());
// then parameter type
t = tf.constructType(m.getParameterTypes()[0]);
assertEquals(CharSequence.class, t.getRawClass());
t = tf.constructType(m.getGenericParameterTypes()[0]);
assertEquals(CharSequence.class, t.getRawClass());
} | com.fasterxml.jackson.databind.type.TestJavaType::testLocalType728 | src/test/java/com/fasterxml/jackson/databind/type/TestJavaType.java | 49 | src/test/java/com/fasterxml/jackson/databind/type/TestJavaType.java | testLocalType728 | package com.fasterxml.jackson.databind.type;
import java.lang.reflect.Method;
import java.util.*;
import com.fasterxml.jackson.databind.BaseMapTest;
import com.fasterxml.jackson.databind.JavaType;
/**
* Simple tests to verify that {@link JavaType} types work to
* some degree
*/
public class TestJavaType
extends BaseMapTest
{
static class BaseType { }
static class SubType extends BaseType { }
static enum MyEnum { A, B; }
static enum MyEnum2 {
A(1), B(2);
private MyEnum2(int value) { }
}
// [databind#728]
static class Issue728 {
public <C extends CharSequence> C method(C input) { return null; }
}
public void testLocalType728() throws Exception
{
TypeFactory tf = TypeFactory.defaultInstance();
Method m = Issue728.class.getMethod("method", CharSequence.class);
assertNotNull(m);
// Start with return type
// first type-erased
JavaType t = tf.constructType(m.getReturnType());
assertEquals(CharSequence.class, t.getRawClass());
// then generic
t = tf.constructType(m.getGenericReturnType());
assertEquals(CharSequence.class, t.getRawClass());
// then parameter type
t = tf.constructType(m.getParameterTypes()[0]);
assertEquals(CharSequence.class, t.getRawClass());
t = tf.constructType(m.getGenericParameterTypes()[0]);
assertEquals(CharSequence.class, t.getRawClass());
}
/*
/**********************************************************
/* Test methods
/**********************************************************
*/
public void testSimpleClass()
{
TypeFactory tf = TypeFactory.defaultInstance();
JavaType baseType = tf.constructType(BaseType.class);
assertSame(BaseType.class, baseType.getRawClass());
assertTrue(baseType.hasRawClass(BaseType.class));
assertFalse(baseType.isArrayType());
assertFalse(baseType.isContainerType());
assertFalse(baseType.isEnumType());
assertFalse(baseType.isInterface());
assertFalse(baseType.isPrimitive());
assertNull(baseType.getContentType());
assertNull(baseType.getValueHandler());
/* both narrow and widen just return type itself (exact, not just
* equal)
* (also note that widen/narrow wouldn't work on basic simple
* class type otherwise)
*/
assertSame(baseType, baseType.narrowBy(BaseType.class));
assertSame(baseType, baseType.widenBy(BaseType.class));
// Also: no narrowing for simple types (but should there be?)
try {
baseType.narrowBy(SubType.class);
} catch (IllegalArgumentException e) {
verifyException(e, "should never be called");
}
// Also, let's try assigning bogus handler
/*
baseType.setValueHandler("xyz"); // untyped
assertEquals("xyz", baseType.getValueHandler());
// illegal to re-set
try {
baseType.setValueHandler("foobar");
fail("Shouldn't allow re-setting value handler");
} catch (IllegalStateException iae) {
verifyException(iae, "Trying to reset");
}
*/
}
public void testMapType()
{
TypeFactory tf = TypeFactory.defaultInstance();
JavaType keyT = tf.constructType(String.class);
JavaType baseT = tf.constructType(BaseType.class);
MapType mapT = MapType.construct(Map.class, keyT, baseT);
assertNotNull(mapT);
assertTrue(mapT.isContainerType());
// NOPs:
assertSame(mapT, mapT.narrowContentsBy(BaseType.class));
assertSame(mapT, mapT.narrowKey(String.class));
assertTrue(mapT.equals(mapT));
assertFalse(mapT.equals(null));
assertFalse(mapT.equals("xyz"));
MapType mapT2 = MapType.construct(HashMap.class, keyT, baseT);
assertFalse(mapT.equals(mapT2));
// Also, must use map type constructor, not simple...
try {
SimpleType.construct(HashMap.class);
} catch (IllegalArgumentException e) {
verifyException(e, "for a Map");
}
}
public void testArrayType()
{
TypeFactory tf = TypeFactory.defaultInstance();
JavaType arrayT = ArrayType.construct(tf.constructType(String.class), null, null);
assertNotNull(arrayT);
assertTrue(arrayT.isContainerType());
// NOPs:
assertSame(arrayT, arrayT.narrowContentsBy(String.class));
assertNotNull(arrayT.toString());
assertTrue(arrayT.equals(arrayT));
assertFalse(arrayT.equals(null));
assertFalse(arrayT.equals("xyz"));
assertTrue(arrayT.equals(ArrayType.construct(tf.constructType(String.class), null, null)));
assertFalse(arrayT.equals(ArrayType.construct(tf.constructType(Integer.class), null, null)));
// Also, must NOT try to create using simple type
try {
SimpleType.construct(String[].class);
} catch (IllegalArgumentException e) {
verifyException(e, "for an array");
}
}
public void testCollectionType()
{
TypeFactory tf = TypeFactory.defaultInstance();
// List<String>
JavaType collectionT = CollectionType.construct(List.class, tf.constructType(String.class));
assertNotNull(collectionT);
assertTrue(collectionT.isContainerType());
// NOPs:
assertSame(collectionT, collectionT.narrowContentsBy(String.class));
assertNotNull(collectionT.toString());
assertTrue(collectionT.equals(collectionT));
assertFalse(collectionT.equals(null));
assertFalse(collectionT.equals("xyz"));
assertTrue(collectionT.equals(CollectionType.construct(List.class, tf.constructType(String.class))));
assertFalse(collectionT.equals(CollectionType.construct(Set.class, tf.constructType(String.class))));
// Also, must NOT try to create using simple type
try {
SimpleType.construct(ArrayList.class);
} catch (IllegalArgumentException e) {
verifyException(e, "for a Collection");
}
}
public void testEnumType()
{
TypeFactory tf = TypeFactory.defaultInstance();
assertTrue(tf.constructType(MyEnum.class).isEnumType());
assertTrue(tf.constructType(MyEnum2.class).isEnumType());
assertTrue(tf.constructType(MyEnum.A.getClass()).isEnumType());
assertTrue(tf.constructType(MyEnum2.A.getClass()).isEnumType());
}
public void testClassKey()
{
ClassKey key = new ClassKey(String.class);
assertEquals(0, key.compareTo(key));
assertTrue(key.equals(key));
assertFalse(key.equals(null));
assertFalse(key.equals("foo"));
assertFalse(key.equals(new ClassKey(Integer.class)));
assertEquals(String.class.getName(), key.toString());
}
// [Issue#116]
public void testJavaTypeAsJLRType()
{
TypeFactory tf = TypeFactory.defaultInstance();
JavaType t1 = tf.constructType(getClass());
// should just get it back as-is:
JavaType t2 = tf.constructType(t1);
assertSame(t1, t2);
}
}
| // You are a professional Java test case writer, please create a test case named `testLocalType728` for the issue `JacksonDatabind-609`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonDatabind-609
//
// ## Issue-Title:
// Problem resolving locally declared generic type
//
// ## Issue-Description:
// (reported by Hal H)
//
//
// Case like:
//
//
//
// ```
// class Something {
// public <T extends Ruleform> T getEntity()
// public <T extends Ruleform> void setEntity(T entity)
// }
// ```
//
// appears to fail on deserialization.
//
//
//
//
public void testLocalType728() throws Exception {
| 49 | 11 | 30 | src/test/java/com/fasterxml/jackson/databind/type/TestJavaType.java | src/test/java | ```markdown
## Issue-ID: JacksonDatabind-609
## Issue-Title:
Problem resolving locally declared generic type
## Issue-Description:
(reported by Hal H)
Case like:
```
class Something {
public <T extends Ruleform> T getEntity()
public <T extends Ruleform> void setEntity(T entity)
}
```
appears to fail on deserialization.
```
You are a professional Java test case writer, please create a test case named `testLocalType728` for the issue `JacksonDatabind-609`, utilizing the provided issue report information and the following function signature.
```java
public void testLocalType728() throws Exception {
```
| 30 | [
"com.fasterxml.jackson.databind.type.TypeFactory"
] | 89ecd6d9cc821a02dff5fac7d139a10f25b7640e9ee5bbc8fbc0440e9d1dd5b6 | public void testLocalType728() throws Exception
| // You are a professional Java test case writer, please create a test case named `testLocalType728` for the issue `JacksonDatabind-609`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonDatabind-609
//
// ## Issue-Title:
// Problem resolving locally declared generic type
//
// ## Issue-Description:
// (reported by Hal H)
//
//
// Case like:
//
//
//
// ```
// class Something {
// public <T extends Ruleform> T getEntity()
// public <T extends Ruleform> void setEntity(T entity)
// }
// ```
//
// appears to fail on deserialization.
//
//
//
//
| JacksonDatabind | package com.fasterxml.jackson.databind.type;
import java.lang.reflect.Method;
import java.util.*;
import com.fasterxml.jackson.databind.BaseMapTest;
import com.fasterxml.jackson.databind.JavaType;
/**
* Simple tests to verify that {@link JavaType} types work to
* some degree
*/
public class TestJavaType
extends BaseMapTest
{
static class BaseType { }
static class SubType extends BaseType { }
static enum MyEnum { A, B; }
static enum MyEnum2 {
A(1), B(2);
private MyEnum2(int value) { }
}
// [databind#728]
static class Issue728 {
public <C extends CharSequence> C method(C input) { return null; }
}
public void testLocalType728() throws Exception
{
TypeFactory tf = TypeFactory.defaultInstance();
Method m = Issue728.class.getMethod("method", CharSequence.class);
assertNotNull(m);
// Start with return type
// first type-erased
JavaType t = tf.constructType(m.getReturnType());
assertEquals(CharSequence.class, t.getRawClass());
// then generic
t = tf.constructType(m.getGenericReturnType());
assertEquals(CharSequence.class, t.getRawClass());
// then parameter type
t = tf.constructType(m.getParameterTypes()[0]);
assertEquals(CharSequence.class, t.getRawClass());
t = tf.constructType(m.getGenericParameterTypes()[0]);
assertEquals(CharSequence.class, t.getRawClass());
}
/*
/**********************************************************
/* Test methods
/**********************************************************
*/
public void testSimpleClass()
{
TypeFactory tf = TypeFactory.defaultInstance();
JavaType baseType = tf.constructType(BaseType.class);
assertSame(BaseType.class, baseType.getRawClass());
assertTrue(baseType.hasRawClass(BaseType.class));
assertFalse(baseType.isArrayType());
assertFalse(baseType.isContainerType());
assertFalse(baseType.isEnumType());
assertFalse(baseType.isInterface());
assertFalse(baseType.isPrimitive());
assertNull(baseType.getContentType());
assertNull(baseType.getValueHandler());
/* both narrow and widen just return type itself (exact, not just
* equal)
* (also note that widen/narrow wouldn't work on basic simple
* class type otherwise)
*/
assertSame(baseType, baseType.narrowBy(BaseType.class));
assertSame(baseType, baseType.widenBy(BaseType.class));
// Also: no narrowing for simple types (but should there be?)
try {
baseType.narrowBy(SubType.class);
} catch (IllegalArgumentException e) {
verifyException(e, "should never be called");
}
// Also, let's try assigning bogus handler
/*
baseType.setValueHandler("xyz"); // untyped
assertEquals("xyz", baseType.getValueHandler());
// illegal to re-set
try {
baseType.setValueHandler("foobar");
fail("Shouldn't allow re-setting value handler");
} catch (IllegalStateException iae) {
verifyException(iae, "Trying to reset");
}
*/
}
public void testMapType()
{
TypeFactory tf = TypeFactory.defaultInstance();
JavaType keyT = tf.constructType(String.class);
JavaType baseT = tf.constructType(BaseType.class);
MapType mapT = MapType.construct(Map.class, keyT, baseT);
assertNotNull(mapT);
assertTrue(mapT.isContainerType());
// NOPs:
assertSame(mapT, mapT.narrowContentsBy(BaseType.class));
assertSame(mapT, mapT.narrowKey(String.class));
assertTrue(mapT.equals(mapT));
assertFalse(mapT.equals(null));
assertFalse(mapT.equals("xyz"));
MapType mapT2 = MapType.construct(HashMap.class, keyT, baseT);
assertFalse(mapT.equals(mapT2));
// Also, must use map type constructor, not simple...
try {
SimpleType.construct(HashMap.class);
} catch (IllegalArgumentException e) {
verifyException(e, "for a Map");
}
}
public void testArrayType()
{
TypeFactory tf = TypeFactory.defaultInstance();
JavaType arrayT = ArrayType.construct(tf.constructType(String.class), null, null);
assertNotNull(arrayT);
assertTrue(arrayT.isContainerType());
// NOPs:
assertSame(arrayT, arrayT.narrowContentsBy(String.class));
assertNotNull(arrayT.toString());
assertTrue(arrayT.equals(arrayT));
assertFalse(arrayT.equals(null));
assertFalse(arrayT.equals("xyz"));
assertTrue(arrayT.equals(ArrayType.construct(tf.constructType(String.class), null, null)));
assertFalse(arrayT.equals(ArrayType.construct(tf.constructType(Integer.class), null, null)));
// Also, must NOT try to create using simple type
try {
SimpleType.construct(String[].class);
} catch (IllegalArgumentException e) {
verifyException(e, "for an array");
}
}
public void testCollectionType()
{
TypeFactory tf = TypeFactory.defaultInstance();
// List<String>
JavaType collectionT = CollectionType.construct(List.class, tf.constructType(String.class));
assertNotNull(collectionT);
assertTrue(collectionT.isContainerType());
// NOPs:
assertSame(collectionT, collectionT.narrowContentsBy(String.class));
assertNotNull(collectionT.toString());
assertTrue(collectionT.equals(collectionT));
assertFalse(collectionT.equals(null));
assertFalse(collectionT.equals("xyz"));
assertTrue(collectionT.equals(CollectionType.construct(List.class, tf.constructType(String.class))));
assertFalse(collectionT.equals(CollectionType.construct(Set.class, tf.constructType(String.class))));
// Also, must NOT try to create using simple type
try {
SimpleType.construct(ArrayList.class);
} catch (IllegalArgumentException e) {
verifyException(e, "for a Collection");
}
}
public void testEnumType()
{
TypeFactory tf = TypeFactory.defaultInstance();
assertTrue(tf.constructType(MyEnum.class).isEnumType());
assertTrue(tf.constructType(MyEnum2.class).isEnumType());
assertTrue(tf.constructType(MyEnum.A.getClass()).isEnumType());
assertTrue(tf.constructType(MyEnum2.A.getClass()).isEnumType());
}
public void testClassKey()
{
ClassKey key = new ClassKey(String.class);
assertEquals(0, key.compareTo(key));
assertTrue(key.equals(key));
assertFalse(key.equals(null));
assertFalse(key.equals("foo"));
assertFalse(key.equals(new ClassKey(Integer.class)));
assertEquals(String.class.getName(), key.toString());
}
// [Issue#116]
public void testJavaTypeAsJLRType()
{
TypeFactory tf = TypeFactory.defaultInstance();
JavaType t1 = tf.constructType(getClass());
// should just get it back as-is:
JavaType t2 = tf.constructType(t1);
assertSame(t1, t2);
}
}
|
||
public void testExtremeValues() throws Exception {
NormalDistribution distribution = (NormalDistribution) getDistribution();
distribution.setMean(0);
distribution.setStandardDeviation(1);
for (int i = 0; i < 100; i+=5) { // make sure no convergence exception
double lowerTail = distribution.cumulativeProbability((double)-i);
double upperTail = distribution.cumulativeProbability((double) i);
if (i < 10) { // make sure not top-coded
assertTrue(lowerTail > 0.0d);
assertTrue(upperTail < 1.0d);
}
else { // make sure top coding not reversed
assertTrue(lowerTail < 0.00001);
assertTrue(upperTail > 0.99999);
}
}
} | org.apache.commons.math.distribution.NormalDistributionTest::testExtremeValues | src/test/org/apache/commons/math/distribution/NormalDistributionTest.java | 143 | src/test/org/apache/commons/math/distribution/NormalDistributionTest.java | testExtremeValues | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.math.distribution;
/**
* Test cases for NormalDistribution.
* Extends ContinuousDistributionAbstractTest. See class javadoc for
* ContinuousDistributionAbstractTest for details.
*
* @version $Revision$ $Date$
*/
public class NormalDistributionTest extends ContinuousDistributionAbstractTest {
/**
* Constructor for NormalDistributionTest.
* @param arg0
*/
public NormalDistributionTest(String arg0) {
super(arg0);
}
//-------------- Implementations for abstract methods -----------------------
/** Creates the default continuous distribution instance to use in tests. */
public ContinuousDistribution makeDistribution() {
return new NormalDistributionImpl(2.1, 1.4);
}
/** Creates the default cumulative probability distribution test input values */
public double[] makeCumulativeTestPoints() {
// quantiles computed using R
return new double[] {-2.226325d, -1.156887d, -0.6439496d, -0.2027951d, 0.3058278d,
6.426325d, 5.356887d, 4.84395d, 4.402795d, 3.894172d};
}
/** Creates the default cumulative probability density test expected values */
public double[] makeCumulativeTestValues() {
return new double[] {0.001d, 0.01d, 0.025d, 0.05d, 0.1d, 0.999d,
0.990d, 0.975d, 0.950d, 0.900d};
}
// --------------------- Override tolerance --------------
protected void setup() throws Exception {
super.setUp();
setTolerance(1E-6);
}
//---------------------------- Additional test cases -------------------------
private void verifyQuantiles() throws Exception {
NormalDistribution distribution = (NormalDistribution) getDistribution();
double mu = distribution.getMean();
double sigma = distribution.getStandardDeviation();
setCumulativeTestPoints( new double[] {mu - 2 *sigma, mu - sigma,
mu, mu + sigma, mu +2 * sigma, mu +3 * sigma, mu + 4 * sigma,
mu + 5 * sigma});
// Quantiles computed using R (same as Mathematica)
setCumulativeTestValues(new double[] {0.02275013, 0.1586553, 0.5, 0.8413447,
0.9772499, 0.9986501, 0.9999683, 0.9999997});
verifyCumulativeProbabilities();
}
public void testQuantiles() throws Exception {
verifyQuantiles();
setDistribution(new NormalDistributionImpl(0, 1));
verifyQuantiles();
setDistribution(new NormalDistributionImpl(0, 0.1));
verifyQuantiles();
}
public void testInverseCumulativeProbabilityExtremes() throws Exception {
setInverseCumulativeTestPoints(new double[] {0, 1});
setInverseCumulativeTestValues(
new double[] {Double.NEGATIVE_INFINITY, Double.POSITIVE_INFINITY});
verifyInverseCumulativeProbabilities();
}
public void testGetMean() {
NormalDistribution distribution = (NormalDistribution) getDistribution();
assertEquals(2.1, distribution.getMean(), 0);
}
public void testSetMean() throws Exception {
double mu = Math.random();
NormalDistribution distribution = (NormalDistribution) getDistribution();
distribution.setMean(mu);
verifyQuantiles();
}
public void testGetStandardDeviation() {
NormalDistribution distribution = (NormalDistribution) getDistribution();
assertEquals(1.4, distribution.getStandardDeviation(), 0);
}
public void testSetStandardDeviation() throws Exception {
double sigma = 0.1d + Math.random();
NormalDistribution distribution = (NormalDistribution) getDistribution();
distribution.setStandardDeviation(sigma);
assertEquals(sigma, distribution.getStandardDeviation(), 0);
verifyQuantiles();
try {
distribution.setStandardDeviation(0);
fail("Expecting IllegalArgumentException for sd = 0");
} catch (IllegalArgumentException ex) {
// Expected
}
}
/**
* Check to make sure top-coding of extreme values works correctly.
* Verifies fix for JIRA MATH-167
*/
public void testExtremeValues() throws Exception {
NormalDistribution distribution = (NormalDistribution) getDistribution();
distribution.setMean(0);
distribution.setStandardDeviation(1);
for (int i = 0; i < 100; i+=5) { // make sure no convergence exception
double lowerTail = distribution.cumulativeProbability((double)-i);
double upperTail = distribution.cumulativeProbability((double) i);
if (i < 10) { // make sure not top-coded
assertTrue(lowerTail > 0.0d);
assertTrue(upperTail < 1.0d);
}
else { // make sure top coding not reversed
assertTrue(lowerTail < 0.00001);
assertTrue(upperTail > 0.99999);
}
}
}
} | // You are a professional Java test case writer, please create a test case named `testExtremeValues` for the issue `Math-MATH-167`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Math-MATH-167
//
// ## Issue-Title:
// ConvergenceException in normal CDF
//
// ## Issue-Description:
//
// NormalDistributionImpl::cumulativeProbability(double x) throws ConvergenceException
//
// if x deviates too much from the mean. For example, when x=+/-100, mean=0, sd=1.
//
// Of course the value of the CDF is hard to evaluate in these cases,
//
// but effectively it should be either zero or one.
//
//
//
//
//
public void testExtremeValues() throws Exception {
| 143 | /**
* Check to make sure top-coding of extreme values works correctly.
* Verifies fix for JIRA MATH-167
*/ | 103 | 127 | src/test/org/apache/commons/math/distribution/NormalDistributionTest.java | src/test | ```markdown
## Issue-ID: Math-MATH-167
## Issue-Title:
ConvergenceException in normal CDF
## Issue-Description:
NormalDistributionImpl::cumulativeProbability(double x) throws ConvergenceException
if x deviates too much from the mean. For example, when x=+/-100, mean=0, sd=1.
Of course the value of the CDF is hard to evaluate in these cases,
but effectively it should be either zero or one.
```
You are a professional Java test case writer, please create a test case named `testExtremeValues` for the issue `Math-MATH-167`, utilizing the provided issue report information and the following function signature.
```java
public void testExtremeValues() throws Exception {
```
| 127 | [
"org.apache.commons.math.distribution.NormalDistributionImpl"
] | 8a79fbfc1f9484718aae5e74d1e3bdc60655d1e82d7a22cc256e70fc3417dde7 | public void testExtremeValues() throws Exception | // You are a professional Java test case writer, please create a test case named `testExtremeValues` for the issue `Math-MATH-167`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Math-MATH-167
//
// ## Issue-Title:
// ConvergenceException in normal CDF
//
// ## Issue-Description:
//
// NormalDistributionImpl::cumulativeProbability(double x) throws ConvergenceException
//
// if x deviates too much from the mean. For example, when x=+/-100, mean=0, sd=1.
//
// Of course the value of the CDF is hard to evaluate in these cases,
//
// but effectively it should be either zero or one.
//
//
//
//
//
| Math | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.math.distribution;
/**
* Test cases for NormalDistribution.
* Extends ContinuousDistributionAbstractTest. See class javadoc for
* ContinuousDistributionAbstractTest for details.
*
* @version $Revision$ $Date$
*/
public class NormalDistributionTest extends ContinuousDistributionAbstractTest {
/**
* Constructor for NormalDistributionTest.
* @param arg0
*/
public NormalDistributionTest(String arg0) {
super(arg0);
}
//-------------- Implementations for abstract methods -----------------------
/** Creates the default continuous distribution instance to use in tests. */
public ContinuousDistribution makeDistribution() {
return new NormalDistributionImpl(2.1, 1.4);
}
/** Creates the default cumulative probability distribution test input values */
public double[] makeCumulativeTestPoints() {
// quantiles computed using R
return new double[] {-2.226325d, -1.156887d, -0.6439496d, -0.2027951d, 0.3058278d,
6.426325d, 5.356887d, 4.84395d, 4.402795d, 3.894172d};
}
/** Creates the default cumulative probability density test expected values */
public double[] makeCumulativeTestValues() {
return new double[] {0.001d, 0.01d, 0.025d, 0.05d, 0.1d, 0.999d,
0.990d, 0.975d, 0.950d, 0.900d};
}
// --------------------- Override tolerance --------------
protected void setup() throws Exception {
super.setUp();
setTolerance(1E-6);
}
//---------------------------- Additional test cases -------------------------
private void verifyQuantiles() throws Exception {
NormalDistribution distribution = (NormalDistribution) getDistribution();
double mu = distribution.getMean();
double sigma = distribution.getStandardDeviation();
setCumulativeTestPoints( new double[] {mu - 2 *sigma, mu - sigma,
mu, mu + sigma, mu +2 * sigma, mu +3 * sigma, mu + 4 * sigma,
mu + 5 * sigma});
// Quantiles computed using R (same as Mathematica)
setCumulativeTestValues(new double[] {0.02275013, 0.1586553, 0.5, 0.8413447,
0.9772499, 0.9986501, 0.9999683, 0.9999997});
verifyCumulativeProbabilities();
}
public void testQuantiles() throws Exception {
verifyQuantiles();
setDistribution(new NormalDistributionImpl(0, 1));
verifyQuantiles();
setDistribution(new NormalDistributionImpl(0, 0.1));
verifyQuantiles();
}
public void testInverseCumulativeProbabilityExtremes() throws Exception {
setInverseCumulativeTestPoints(new double[] {0, 1});
setInverseCumulativeTestValues(
new double[] {Double.NEGATIVE_INFINITY, Double.POSITIVE_INFINITY});
verifyInverseCumulativeProbabilities();
}
public void testGetMean() {
NormalDistribution distribution = (NormalDistribution) getDistribution();
assertEquals(2.1, distribution.getMean(), 0);
}
public void testSetMean() throws Exception {
double mu = Math.random();
NormalDistribution distribution = (NormalDistribution) getDistribution();
distribution.setMean(mu);
verifyQuantiles();
}
public void testGetStandardDeviation() {
NormalDistribution distribution = (NormalDistribution) getDistribution();
assertEquals(1.4, distribution.getStandardDeviation(), 0);
}
public void testSetStandardDeviation() throws Exception {
double sigma = 0.1d + Math.random();
NormalDistribution distribution = (NormalDistribution) getDistribution();
distribution.setStandardDeviation(sigma);
assertEquals(sigma, distribution.getStandardDeviation(), 0);
verifyQuantiles();
try {
distribution.setStandardDeviation(0);
fail("Expecting IllegalArgumentException for sd = 0");
} catch (IllegalArgumentException ex) {
// Expected
}
}
/**
* Check to make sure top-coding of extreme values works correctly.
* Verifies fix for JIRA MATH-167
*/
public void testExtremeValues() throws Exception {
NormalDistribution distribution = (NormalDistribution) getDistribution();
distribution.setMean(0);
distribution.setStandardDeviation(1);
for (int i = 0; i < 100; i+=5) { // make sure no convergence exception
double lowerTail = distribution.cumulativeProbability((double)-i);
double upperTail = distribution.cumulativeProbability((double) i);
if (i < 10) { // make sure not top-coded
assertTrue(lowerTail > 0.0d);
assertTrue(upperTail < 1.0d);
}
else { // make sure top coding not reversed
assertTrue(lowerTail < 0.00001);
assertTrue(upperTail > 0.99999);
}
}
}
} |
|
public void testArrayWithDefaultTyping() throws Exception
{
ObjectMapper mapper = new ObjectMapper()
.enableDefaultTyping();
JsonNode array = mapper.readTree("[ 1, 2 ]");
assertTrue(array.isArray());
assertEquals(2, array.size());
JsonNode obj = mapper.readTree("{ \"a\" : 2 }");
assertTrue(obj.isObject());
assertEquals(1, obj.size());
assertEquals(2, obj.path("a").asInt());
} | com.fasterxml.jackson.databind.node.TestJsonNode::testArrayWithDefaultTyping | src/test/java/com/fasterxml/jackson/databind/node/TestJsonNode.java | 121 | src/test/java/com/fasterxml/jackson/databind/node/TestJsonNode.java | testArrayWithDefaultTyping | package com.fasterxml.jackson.databind.node;
import com.fasterxml.jackson.core.*;
import com.fasterxml.jackson.databind.*;
/**
* Basic tests for {@link JsonNode} base class and some features
* of implementation classes
*/
public class TestJsonNode extends NodeTestBase
{
public void testText()
{
assertNull(TextNode.valueOf(null));
TextNode empty = TextNode.valueOf("");
assertStandardEquals(empty);
assertSame(TextNode.EMPTY_STRING_NODE, empty);
// 1.6:
assertNodeNumbers(TextNode.valueOf("-3"), -3, -3.0);
assertNodeNumbers(TextNode.valueOf("17.75"), 17, 17.75);
// [JACKSON-587]
long value = 127353264013893L;
TextNode n = TextNode.valueOf(String.valueOf(value));
assertEquals(value, n.asLong());
// and then with non-numeric input
n = TextNode.valueOf("foobar");
assertNodeNumbersForNonNumeric(n);
assertEquals("foobar", n.asText("barf"));
assertEquals("", empty.asText("xyz"));
assertTrue(TextNode.valueOf("true").asBoolean(true));
assertTrue(TextNode.valueOf("true").asBoolean(false));
assertFalse(TextNode.valueOf("false").asBoolean(true));
assertFalse(TextNode.valueOf("false").asBoolean(false));
}
public void testBoolean()
{
BooleanNode f = BooleanNode.getFalse();
assertNotNull(f);
assertTrue(f.isBoolean());
assertSame(f, BooleanNode.valueOf(false));
assertStandardEquals(f);
assertFalse(f.booleanValue());
assertFalse(f.asBoolean());
assertEquals("false", f.asText());
assertEquals(JsonToken.VALUE_FALSE, f.asToken());
// and ditto for true
BooleanNode t = BooleanNode.getTrue();
assertNotNull(t);
assertTrue(t.isBoolean());
assertSame(t, BooleanNode.valueOf(true));
assertStandardEquals(t);
assertTrue(t.booleanValue());
assertTrue(t.asBoolean());
assertEquals("true", t.asText());
assertEquals(JsonToken.VALUE_TRUE, t.asToken());
// 1.6:
assertNodeNumbers(f, 0, 0.0);
assertNodeNumbers(t, 1, 1.0);
}
public void testBinary() throws Exception
{
assertNull(BinaryNode.valueOf(null));
assertNull(BinaryNode.valueOf(null, 0, 0));
BinaryNode empty = BinaryNode.valueOf(new byte[1], 0, 0);
assertSame(BinaryNode.EMPTY_BINARY_NODE, empty);
assertStandardEquals(empty);
byte[] data = new byte[3];
data[1] = (byte) 3;
BinaryNode n = BinaryNode.valueOf(data, 1, 1);
data[2] = (byte) 3;
BinaryNode n2 = BinaryNode.valueOf(data, 2, 1);
assertTrue(n.equals(n2));
assertEquals("\"Aw==\"", n.toString());
assertEquals("AAMD", new BinaryNode(data).asText());
assertNodeNumbersForNonNumeric(n);
}
public void testPOJO()
{
POJONode n = new POJONode("x"); // not really a pojo but that's ok
assertStandardEquals(n);
assertEquals(n, new POJONode("x"));
assertEquals("x", n.asText());
// not sure if this is what it'll remain as but:
assertEquals("x", n.toString());
assertEquals(new POJONode(null), new POJONode(null));
// default; non-numeric
assertNodeNumbersForNonNumeric(n);
// but if wrapping actual number, use it
assertNodeNumbers(new POJONode(Integer.valueOf(123)), 123, 123.0);
}
// [databind#793]
public void testArrayWithDefaultTyping() throws Exception
{
ObjectMapper mapper = new ObjectMapper()
.enableDefaultTyping();
JsonNode array = mapper.readTree("[ 1, 2 ]");
assertTrue(array.isArray());
assertEquals(2, array.size());
JsonNode obj = mapper.readTree("{ \"a\" : 2 }");
assertTrue(obj.isObject());
assertEquals(1, obj.size());
assertEquals(2, obj.path("a").asInt());
}
} | // You are a professional Java test case writer, please create a test case named `testArrayWithDefaultTyping` for the issue `JacksonDatabind-793`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonDatabind-793
//
// ## Issue-Title:
// readTree does not work with defaultTyping enabled but no type info provided
//
// ## Issue-Description:
// I have enabled `defaultTyping`, and serialized `Foo` entity with no type info. I'm trying to read json as a tree with `mapper.readTree(json)`, and it throws an exception
//
//
//
// ```
// Exception in thread "main" com.fasterxml.jackson.databind.JsonMappingException:
// Unexpected token (START\_OBJECT), expected START\_ARRAY: need JSON Array to contain As.WRAPPER\_ARRAY
// type information for class com.fasterxml.jackson.databind.JsonNode
// at [Source: {
// "bar" : "bar"
// }; line: 1, column: 1]
// at com.fasterxml.jackson.databind.JsonMappingException.from(JsonMappingException.java:148)
// at com.fasterxml.jackson.databind.DeserializationContext.wrongTokenException(DeserializationContext.java:927)
// at com.fasterxml.jackson.databind.jsontype.impl.AsArrayTypeDeserializer.\_locateTypeId(AsArrayTypeDeserializer.java:127)
// at com.fasterxml.jackson.databind.jsontype.impl.AsArrayTypeDeserializer.\_deserialize(AsArrayTypeDeserializer.java:93)
// at com.fasterxml.jackson.databind.jsontype.impl.AsArrayTypeDeserializer.deserializeTypedFromAny(AsArrayTypeDeserializer.java:68)
// at com.fasterxml.jackson.databind.deser.std.BaseNodeDeserializer.deserializeWithType(JsonNodeDeserializer.java:144)
// at com.fasterxml.jackson.databind.deser.std.JsonNodeDeserializer.deserializeWithType(JsonNodeDeserializer.java:14)
// at com.fasterxml.jackson.databind.deser.impl.TypeWrappedDeserializer.deserialize(TypeWrappedDeserializer.java:42)
// at com.fasterxml.jackson.databind.ObjectMapper.\_readMapAndClose(ObjectMapper.java:3562)
// at com.fasterxml.jackson.databind.ObjectMapper.readTree(ObjectMapper.java:2136)
// at test.App.main(App.java:23)
// ```
//
// However, if I disable `defaultTyping`, the same code works fine. So, `readTree(json)` does not actually need type info for the root element, because it works when `defaultTyping` is disabled (i.e. `{"bar" : "bar"}`), but it throws the exception when `defaultTyping` is enabled, that's why it looks like a bug. The same thing happens for `valueToTree(foo)`.
//
// Jackson version is `2.5.3`
//
// Full code is provided.
//
//
//
// ```
// import com.fasterxml.jackson.databind.JsonNode;
// import com.fasterxml.jackson.databind.MapperFeature;
// import com.fasterxml.jackson.databind.ObjectMapper;
// import com.fasterxml.jackson.databind.SerializationFeature;
// import java.io.IOException;
//
// public class App {
// public static void main(String[] args) throws IOException {
// ObjectMapper mapper = new ObjectMapper()
// .enableDefaultTyping() // works fine with disableDefaultTyping()
// .enable(MapperFeature.AUTO\_DETECT\_GETTERS)
// .enable(MapperFeature.REQUIRE\_SETTERS\_FOR\_GETTERS)
//
public void testArrayWithDefaultTyping() throws Exception {
| 121 | // [databind#793] | 17 | 108 | src/test/java/com/fasterxml/jackson/databind/node/TestJsonNode.java | src/test/java | ```markdown
## Issue-ID: JacksonDatabind-793
## Issue-Title:
readTree does not work with defaultTyping enabled but no type info provided
## Issue-Description:
I have enabled `defaultTyping`, and serialized `Foo` entity with no type info. I'm trying to read json as a tree with `mapper.readTree(json)`, and it throws an exception
```
Exception in thread "main" com.fasterxml.jackson.databind.JsonMappingException:
Unexpected token (START\_OBJECT), expected START\_ARRAY: need JSON Array to contain As.WRAPPER\_ARRAY
type information for class com.fasterxml.jackson.databind.JsonNode
at [Source: {
"bar" : "bar"
}; line: 1, column: 1]
at com.fasterxml.jackson.databind.JsonMappingException.from(JsonMappingException.java:148)
at com.fasterxml.jackson.databind.DeserializationContext.wrongTokenException(DeserializationContext.java:927)
at com.fasterxml.jackson.databind.jsontype.impl.AsArrayTypeDeserializer.\_locateTypeId(AsArrayTypeDeserializer.java:127)
at com.fasterxml.jackson.databind.jsontype.impl.AsArrayTypeDeserializer.\_deserialize(AsArrayTypeDeserializer.java:93)
at com.fasterxml.jackson.databind.jsontype.impl.AsArrayTypeDeserializer.deserializeTypedFromAny(AsArrayTypeDeserializer.java:68)
at com.fasterxml.jackson.databind.deser.std.BaseNodeDeserializer.deserializeWithType(JsonNodeDeserializer.java:144)
at com.fasterxml.jackson.databind.deser.std.JsonNodeDeserializer.deserializeWithType(JsonNodeDeserializer.java:14)
at com.fasterxml.jackson.databind.deser.impl.TypeWrappedDeserializer.deserialize(TypeWrappedDeserializer.java:42)
at com.fasterxml.jackson.databind.ObjectMapper.\_readMapAndClose(ObjectMapper.java:3562)
at com.fasterxml.jackson.databind.ObjectMapper.readTree(ObjectMapper.java:2136)
at test.App.main(App.java:23)
```
However, if I disable `defaultTyping`, the same code works fine. So, `readTree(json)` does not actually need type info for the root element, because it works when `defaultTyping` is disabled (i.e. `{"bar" : "bar"}`), but it throws the exception when `defaultTyping` is enabled, that's why it looks like a bug. The same thing happens for `valueToTree(foo)`.
Jackson version is `2.5.3`
Full code is provided.
```
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.MapperFeature;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.SerializationFeature;
import java.io.IOException;
public class App {
public static void main(String[] args) throws IOException {
ObjectMapper mapper = new ObjectMapper()
.enableDefaultTyping() // works fine with disableDefaultTyping()
.enable(MapperFeature.AUTO\_DETECT\_GETTERS)
.enable(MapperFeature.REQUIRE\_SETTERS\_FOR\_GETTERS)
```
You are a professional Java test case writer, please create a test case named `testArrayWithDefaultTyping` for the issue `JacksonDatabind-793`, utilizing the provided issue report information and the following function signature.
```java
public void testArrayWithDefaultTyping() throws Exception {
```
| 108 | [
"com.fasterxml.jackson.databind.ObjectMapper"
] | 8af5c1b559a0b6435ca696c9eb366c43c1e1866eb582c5bd7575e436e7dbde9f | public void testArrayWithDefaultTyping() throws Exception
| // You are a professional Java test case writer, please create a test case named `testArrayWithDefaultTyping` for the issue `JacksonDatabind-793`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonDatabind-793
//
// ## Issue-Title:
// readTree does not work with defaultTyping enabled but no type info provided
//
// ## Issue-Description:
// I have enabled `defaultTyping`, and serialized `Foo` entity with no type info. I'm trying to read json as a tree with `mapper.readTree(json)`, and it throws an exception
//
//
//
// ```
// Exception in thread "main" com.fasterxml.jackson.databind.JsonMappingException:
// Unexpected token (START\_OBJECT), expected START\_ARRAY: need JSON Array to contain As.WRAPPER\_ARRAY
// type information for class com.fasterxml.jackson.databind.JsonNode
// at [Source: {
// "bar" : "bar"
// }; line: 1, column: 1]
// at com.fasterxml.jackson.databind.JsonMappingException.from(JsonMappingException.java:148)
// at com.fasterxml.jackson.databind.DeserializationContext.wrongTokenException(DeserializationContext.java:927)
// at com.fasterxml.jackson.databind.jsontype.impl.AsArrayTypeDeserializer.\_locateTypeId(AsArrayTypeDeserializer.java:127)
// at com.fasterxml.jackson.databind.jsontype.impl.AsArrayTypeDeserializer.\_deserialize(AsArrayTypeDeserializer.java:93)
// at com.fasterxml.jackson.databind.jsontype.impl.AsArrayTypeDeserializer.deserializeTypedFromAny(AsArrayTypeDeserializer.java:68)
// at com.fasterxml.jackson.databind.deser.std.BaseNodeDeserializer.deserializeWithType(JsonNodeDeserializer.java:144)
// at com.fasterxml.jackson.databind.deser.std.JsonNodeDeserializer.deserializeWithType(JsonNodeDeserializer.java:14)
// at com.fasterxml.jackson.databind.deser.impl.TypeWrappedDeserializer.deserialize(TypeWrappedDeserializer.java:42)
// at com.fasterxml.jackson.databind.ObjectMapper.\_readMapAndClose(ObjectMapper.java:3562)
// at com.fasterxml.jackson.databind.ObjectMapper.readTree(ObjectMapper.java:2136)
// at test.App.main(App.java:23)
// ```
//
// However, if I disable `defaultTyping`, the same code works fine. So, `readTree(json)` does not actually need type info for the root element, because it works when `defaultTyping` is disabled (i.e. `{"bar" : "bar"}`), but it throws the exception when `defaultTyping` is enabled, that's why it looks like a bug. The same thing happens for `valueToTree(foo)`.
//
// Jackson version is `2.5.3`
//
// Full code is provided.
//
//
//
// ```
// import com.fasterxml.jackson.databind.JsonNode;
// import com.fasterxml.jackson.databind.MapperFeature;
// import com.fasterxml.jackson.databind.ObjectMapper;
// import com.fasterxml.jackson.databind.SerializationFeature;
// import java.io.IOException;
//
// public class App {
// public static void main(String[] args) throws IOException {
// ObjectMapper mapper = new ObjectMapper()
// .enableDefaultTyping() // works fine with disableDefaultTyping()
// .enable(MapperFeature.AUTO\_DETECT\_GETTERS)
// .enable(MapperFeature.REQUIRE\_SETTERS\_FOR\_GETTERS)
//
| JacksonDatabind | package com.fasterxml.jackson.databind.node;
import com.fasterxml.jackson.core.*;
import com.fasterxml.jackson.databind.*;
/**
* Basic tests for {@link JsonNode} base class and some features
* of implementation classes
*/
public class TestJsonNode extends NodeTestBase
{
public void testText()
{
assertNull(TextNode.valueOf(null));
TextNode empty = TextNode.valueOf("");
assertStandardEquals(empty);
assertSame(TextNode.EMPTY_STRING_NODE, empty);
// 1.6:
assertNodeNumbers(TextNode.valueOf("-3"), -3, -3.0);
assertNodeNumbers(TextNode.valueOf("17.75"), 17, 17.75);
// [JACKSON-587]
long value = 127353264013893L;
TextNode n = TextNode.valueOf(String.valueOf(value));
assertEquals(value, n.asLong());
// and then with non-numeric input
n = TextNode.valueOf("foobar");
assertNodeNumbersForNonNumeric(n);
assertEquals("foobar", n.asText("barf"));
assertEquals("", empty.asText("xyz"));
assertTrue(TextNode.valueOf("true").asBoolean(true));
assertTrue(TextNode.valueOf("true").asBoolean(false));
assertFalse(TextNode.valueOf("false").asBoolean(true));
assertFalse(TextNode.valueOf("false").asBoolean(false));
}
public void testBoolean()
{
BooleanNode f = BooleanNode.getFalse();
assertNotNull(f);
assertTrue(f.isBoolean());
assertSame(f, BooleanNode.valueOf(false));
assertStandardEquals(f);
assertFalse(f.booleanValue());
assertFalse(f.asBoolean());
assertEquals("false", f.asText());
assertEquals(JsonToken.VALUE_FALSE, f.asToken());
// and ditto for true
BooleanNode t = BooleanNode.getTrue();
assertNotNull(t);
assertTrue(t.isBoolean());
assertSame(t, BooleanNode.valueOf(true));
assertStandardEquals(t);
assertTrue(t.booleanValue());
assertTrue(t.asBoolean());
assertEquals("true", t.asText());
assertEquals(JsonToken.VALUE_TRUE, t.asToken());
// 1.6:
assertNodeNumbers(f, 0, 0.0);
assertNodeNumbers(t, 1, 1.0);
}
public void testBinary() throws Exception
{
assertNull(BinaryNode.valueOf(null));
assertNull(BinaryNode.valueOf(null, 0, 0));
BinaryNode empty = BinaryNode.valueOf(new byte[1], 0, 0);
assertSame(BinaryNode.EMPTY_BINARY_NODE, empty);
assertStandardEquals(empty);
byte[] data = new byte[3];
data[1] = (byte) 3;
BinaryNode n = BinaryNode.valueOf(data, 1, 1);
data[2] = (byte) 3;
BinaryNode n2 = BinaryNode.valueOf(data, 2, 1);
assertTrue(n.equals(n2));
assertEquals("\"Aw==\"", n.toString());
assertEquals("AAMD", new BinaryNode(data).asText());
assertNodeNumbersForNonNumeric(n);
}
public void testPOJO()
{
POJONode n = new POJONode("x"); // not really a pojo but that's ok
assertStandardEquals(n);
assertEquals(n, new POJONode("x"));
assertEquals("x", n.asText());
// not sure if this is what it'll remain as but:
assertEquals("x", n.toString());
assertEquals(new POJONode(null), new POJONode(null));
// default; non-numeric
assertNodeNumbersForNonNumeric(n);
// but if wrapping actual number, use it
assertNodeNumbers(new POJONode(Integer.valueOf(123)), 123, 123.0);
}
// [databind#793]
public void testArrayWithDefaultTyping() throws Exception
{
ObjectMapper mapper = new ObjectMapper()
.enableDefaultTyping();
JsonNode array = mapper.readTree("[ 1, 2 ]");
assertTrue(array.isArray());
assertEquals(2, array.size());
JsonNode obj = mapper.readTree("{ \"a\" : 2 }");
assertTrue(obj.isObject());
assertEquals(1, obj.size());
assertEquals(2, obj.path("a").asInt());
}
} |
|
public void testNormalizedStandard_periodType_months1() {
Period test = new Period(1, 15, 0, 0, 0, 0, 0, 0);
Period result = test.normalizedStandard(PeriodType.months());
assertEquals(new Period(1, 15, 0, 0, 0, 0, 0, 0), test);
assertEquals(new Period(0, 27, 0, 0, 0, 0, 0, 0, PeriodType.months()), result);
} | org.joda.time.TestPeriod_Basics::testNormalizedStandard_periodType_months1 | src/test/java/org/joda/time/TestPeriod_Basics.java | 1,524 | src/test/java/org/joda/time/TestPeriod_Basics.java | testNormalizedStandard_periodType_months1 | /*
* Copyright 2001-2005 Stephen Colebourne
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.joda.time;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.math.BigInteger;
import java.util.Arrays;
import java.util.Locale;
import java.util.TimeZone;
import junit.framework.TestCase;
import junit.framework.TestSuite;
import org.joda.time.base.BasePeriod;
import org.joda.time.format.PeriodFormat;
import org.joda.time.format.PeriodFormatter;
/**
* This class is a Junit unit test for Duration.
*
* @author Stephen Colebourne
*/
public class TestPeriod_Basics extends TestCase {
// Test in 2002/03 as time zones are more well known
// (before the late 90's they were all over the place)
//private static final DateTimeZone PARIS = DateTimeZone.forID("Europe/Paris");
private static final DateTimeZone LONDON = DateTimeZone.forID("Europe/London");
long y2002days = 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 +
365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365;
long y2003days = 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 +
365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365 + 365;
// 2002-06-09
private long TEST_TIME_NOW =
(y2002days + 31L + 28L + 31L + 30L + 31L + 9L -1L) * DateTimeConstants.MILLIS_PER_DAY;
// 2002-04-05
private long TEST_TIME1 =
(y2002days + 31L + 28L + 31L + 5L -1L) * DateTimeConstants.MILLIS_PER_DAY
+ 12L * DateTimeConstants.MILLIS_PER_HOUR
+ 24L * DateTimeConstants.MILLIS_PER_MINUTE;
// 2003-05-06
private long TEST_TIME2 =
(y2003days + 31L + 28L + 31L + 30L + 6L -1L) * DateTimeConstants.MILLIS_PER_DAY
+ 14L * DateTimeConstants.MILLIS_PER_HOUR
+ 28L * DateTimeConstants.MILLIS_PER_MINUTE;
private DateTimeZone originalDateTimeZone = null;
private TimeZone originalTimeZone = null;
private Locale originalLocale = null;
public static void main(String[] args) {
junit.textui.TestRunner.run(suite());
}
public static TestSuite suite() {
return new TestSuite(TestPeriod_Basics.class);
}
public TestPeriod_Basics(String name) {
super(name);
}
protected void setUp() throws Exception {
DateTimeUtils.setCurrentMillisFixed(TEST_TIME_NOW);
originalDateTimeZone = DateTimeZone.getDefault();
originalTimeZone = TimeZone.getDefault();
originalLocale = Locale.getDefault();
DateTimeZone.setDefault(LONDON);
TimeZone.setDefault(TimeZone.getTimeZone("Europe/London"));
Locale.setDefault(Locale.UK);
}
protected void tearDown() throws Exception {
DateTimeUtils.setCurrentMillisSystem();
DateTimeZone.setDefault(originalDateTimeZone);
TimeZone.setDefault(originalTimeZone);
Locale.setDefault(originalLocale);
originalDateTimeZone = null;
originalTimeZone = null;
originalLocale = null;
}
//-----------------------------------------------------------------------
public void testTest() {
assertEquals("2002-06-09T00:00:00.000Z", new Instant(TEST_TIME_NOW).toString());
assertEquals("2002-04-05T12:24:00.000Z", new Instant(TEST_TIME1).toString());
assertEquals("2003-05-06T14:28:00.000Z", new Instant(TEST_TIME2).toString());
}
//-----------------------------------------------------------------------
public void testGetPeriodType() {
Period test = new Period(0L);
assertEquals(PeriodType.standard(), test.getPeriodType());
}
public void testGetMethods() {
Period test = new Period(0L);
assertEquals(0, test.getYears());
assertEquals(0, test.getMonths());
assertEquals(0, test.getWeeks());
assertEquals(0, test.getDays());
assertEquals(0, test.getHours());
assertEquals(0, test.getMinutes());
assertEquals(0, test.getSeconds());
assertEquals(0, test.getMillis());
}
public void testValueIndexMethods() {
Period test = new Period(1, 0, 0, 4, 5, 6, 7, 8, PeriodType.yearDayTime());
assertEquals(6, test.size());
assertEquals(1, test.getValue(0));
assertEquals(4, test.getValue(1));
assertEquals(5, test.getValue(2));
assertEquals(6, test.getValue(3));
assertEquals(7, test.getValue(4));
assertEquals(8, test.getValue(5));
assertEquals(true, Arrays.equals(new int[] {1, 4, 5, 6, 7, 8}, test.getValues()));
}
public void testTypeIndexMethods() {
Period test = new Period(1, 0, 0, 4, 5, 6, 7, 8, PeriodType.yearDayTime());
assertEquals(6, test.size());
assertEquals(DurationFieldType.years(), test.getFieldType(0));
assertEquals(DurationFieldType.days(), test.getFieldType(1));
assertEquals(DurationFieldType.hours(), test.getFieldType(2));
assertEquals(DurationFieldType.minutes(), test.getFieldType(3));
assertEquals(DurationFieldType.seconds(), test.getFieldType(4));
assertEquals(DurationFieldType.millis(), test.getFieldType(5));
assertEquals(true, Arrays.equals(new DurationFieldType[] {
DurationFieldType.years(), DurationFieldType.days(), DurationFieldType.hours(),
DurationFieldType.minutes(), DurationFieldType.seconds(), DurationFieldType.millis()},
test.getFieldTypes()));
}
public void testIsSupported() {
Period test = new Period(1, 0, 0, 4, 5, 6, 7, 8, PeriodType.yearDayTime());
assertEquals(true, test.isSupported(DurationFieldType.years()));
assertEquals(false, test.isSupported(DurationFieldType.months()));
assertEquals(false, test.isSupported(DurationFieldType.weeks()));
assertEquals(true, test.isSupported(DurationFieldType.days()));
assertEquals(true, test.isSupported(DurationFieldType.hours()));
assertEquals(true, test.isSupported(DurationFieldType.minutes()));
assertEquals(true, test.isSupported(DurationFieldType.seconds()));
assertEquals(true, test.isSupported(DurationFieldType.millis()));
}
public void testIndexOf() {
Period test = new Period(1, 0, 0, 4, 5, 6, 7, 8, PeriodType.yearDayTime());
assertEquals(0, test.indexOf(DurationFieldType.years()));
assertEquals(-1, test.indexOf(DurationFieldType.months()));
assertEquals(-1, test.indexOf(DurationFieldType.weeks()));
assertEquals(1, test.indexOf(DurationFieldType.days()));
assertEquals(2, test.indexOf(DurationFieldType.hours()));
assertEquals(3, test.indexOf(DurationFieldType.minutes()));
assertEquals(4, test.indexOf(DurationFieldType.seconds()));
assertEquals(5, test.indexOf(DurationFieldType.millis()));
}
public void testGet() {
Period test = new Period(1, 0, 0, 4, 5, 6, 7, 8, PeriodType.yearDayTime());
assertEquals(1, test.get(DurationFieldType.years()));
assertEquals(0, test.get(DurationFieldType.months()));
assertEquals(0, test.get(DurationFieldType.weeks()));
assertEquals(4, test.get(DurationFieldType.days()));
assertEquals(5, test.get(DurationFieldType.hours()));
assertEquals(6, test.get(DurationFieldType.minutes()));
assertEquals(7, test.get(DurationFieldType.seconds()));
assertEquals(8, test.get(DurationFieldType.millis()));
}
public void testEqualsHashCode() {
Period test1 = new Period(123L);
Period test2 = new Period(123L);
assertEquals(true, test1.equals(test2));
assertEquals(true, test2.equals(test1));
assertEquals(true, test1.equals(test1));
assertEquals(true, test2.equals(test2));
assertEquals(true, test1.hashCode() == test2.hashCode());
assertEquals(true, test1.hashCode() == test1.hashCode());
assertEquals(true, test2.hashCode() == test2.hashCode());
Period test3 = new Period(321L);
assertEquals(false, test1.equals(test3));
assertEquals(false, test2.equals(test3));
assertEquals(false, test3.equals(test1));
assertEquals(false, test3.equals(test2));
assertEquals(false, test1.hashCode() == test3.hashCode());
assertEquals(false, test2.hashCode() == test3.hashCode());
assertEquals(false, test1.equals("Hello"));
assertEquals(true, test1.equals(new MockPeriod(123L)));
assertEquals(false, test1.equals(new Period(123L, PeriodType.dayTime())));
}
class MockPeriod extends BasePeriod {
private static final long serialVersionUID = 1L;
public MockPeriod(long value) {
super(value, null, null);
}
}
//-----------------------------------------------------------------------
public void testSerialization() throws Exception {
Period test = new Period(123L);
ByteArrayOutputStream baos = new ByteArrayOutputStream();
ObjectOutputStream oos = new ObjectOutputStream(baos);
oos.writeObject(test);
byte[] bytes = baos.toByteArray();
oos.close();
ByteArrayInputStream bais = new ByteArrayInputStream(bytes);
ObjectInputStream ois = new ObjectInputStream(bais);
Period result = (Period) ois.readObject();
ois.close();
assertEquals(test, result);
}
// //-----------------------------------------------------------------------
// public void testAddTo1() {
// long expected = TEST_TIME_NOW;
// expected = ISOChronology.getInstance().years().add(expected, 1);
// expected = ISOChronology.getInstance().months().add(expected, 2);
// expected = ISOChronology.getInstance().weeks().add(expected, 3);
// expected = ISOChronology.getInstance().days().add(expected, 4);
// expected = ISOChronology.getInstance().hours().add(expected, 5);
// expected = ISOChronology.getInstance().minutes().add(expected, 6);
// expected = ISOChronology.getInstance().seconds().add(expected, 7);
// expected = ISOChronology.getInstance().millis().add(expected, 8);
//
// Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8);
// long added = test.addTo(TEST_TIME_NOW, 1);
// assertEquals(expected, added);
// }
//
// public void testAddTo2() {
// long expected = TEST_TIME_NOW;
// expected = ISOChronology.getInstance().years().add(expected, -2);
// expected = ISOChronology.getInstance().months().add(expected, -4);
// expected = ISOChronology.getInstance().weeks().add(expected, -6);
// expected = ISOChronology.getInstance().days().add(expected, -8);
// expected = ISOChronology.getInstance().hours().add(expected, -10);
// expected = ISOChronology.getInstance().minutes().add(expected, -12);
// expected = ISOChronology.getInstance().seconds().add(expected, -14);
// expected = ISOChronology.getInstance().millis().add(expected, -16);
//
// Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8);
// long added = test.addTo(TEST_TIME_NOW, -2);
// assertEquals(expected, added);
// }
//
// public void testAddTo3() {
// long expected = TEST_TIME_NOW;
// Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8);
// long added = test.addTo(TEST_TIME_NOW, 0);
// assertEquals(expected, added);
// }
//
// public void testAddTo4() {
// long expected = TEST_TIME_NOW + 100L;
// Period test = new Period(100L);
// long added = test.addTo(TEST_TIME_NOW, 1);
// assertEquals(expected, added);
// }
//
// //-----------------------------------------------------------------------
// public void testAddToWithChronology1() {
// long expected = TEST_TIME_NOW;
// expected = ISOChronology.getInstance().years().add(expected, 1);
// expected = ISOChronology.getInstance().months().add(expected, 2);
// expected = ISOChronology.getInstance().weeks().add(expected, 3);
// expected = ISOChronology.getInstance().days().add(expected, 4);
// expected = ISOChronology.getInstance().hours().add(expected, 5);
// expected = ISOChronology.getInstance().minutes().add(expected, 6);
// expected = ISOChronology.getInstance().seconds().add(expected, 7);
// expected = ISOChronology.getInstance().millis().add(expected, 8);
//
// Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8);
// long added = test.addTo(TEST_TIME_NOW, 1, ISOChronology.getInstance());
// assertEquals(expected, added);
// }
//
// public void testAddToWithChronology2() {
// long expected = TEST_TIME_NOW;
// expected = ISOChronology.getInstanceUTC().years().add(expected, -2);
// expected = ISOChronology.getInstanceUTC().months().add(expected, -4);
// expected = ISOChronology.getInstanceUTC().weeks().add(expected, -6);
// expected = ISOChronology.getInstanceUTC().days().add(expected, -8);
// expected = ISOChronology.getInstanceUTC().hours().add(expected, -10);
// expected = ISOChronology.getInstanceUTC().minutes().add(expected, -12);
// expected = ISOChronology.getInstanceUTC().seconds().add(expected, -14);
// expected = ISOChronology.getInstanceUTC().millis().add(expected, -16);
//
// Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8, PeriodType.standard());
// long added = test.addTo(TEST_TIME_NOW, -2, ISOChronology.getInstanceUTC()); // local specified so use it
// assertEquals(expected, added);
// }
//
// public void testAddToWithChronology3() {
// long expected = TEST_TIME_NOW;
// expected = ISOChronology.getInstance().years().add(expected, -2);
// expected = ISOChronology.getInstance().months().add(expected, -4);
// expected = ISOChronology.getInstance().weeks().add(expected, -6);
// expected = ISOChronology.getInstance().days().add(expected, -8);
// expected = ISOChronology.getInstance().hours().add(expected, -10);
// expected = ISOChronology.getInstance().minutes().add(expected, -12);
// expected = ISOChronology.getInstance().seconds().add(expected, -14);
// expected = ISOChronology.getInstance().millis().add(expected, -16);
//
// Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8, PeriodType.standard());
// long added = test.addTo(TEST_TIME_NOW, -2, null); // no chrono specified so use default
// assertEquals(expected, added);
// }
//
// //-----------------------------------------------------------------------
// public void testAddToRI1() {
// long expected = TEST_TIME_NOW;
// expected = ISOChronology.getInstance().years().add(expected, 1);
// expected = ISOChronology.getInstance().months().add(expected, 2);
// expected = ISOChronology.getInstance().weeks().add(expected, 3);
// expected = ISOChronology.getInstance().days().add(expected, 4);
// expected = ISOChronology.getInstance().hours().add(expected, 5);
// expected = ISOChronology.getInstance().minutes().add(expected, 6);
// expected = ISOChronology.getInstance().seconds().add(expected, 7);
// expected = ISOChronology.getInstance().millis().add(expected, 8);
//
// Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8);
// DateTime added = test.addTo(new Instant(), 1); // Instant has no time zone, use default
// assertEquals(expected, added.getMillis());
// assertEquals(ISOChronology.getInstance(), added.getChronology());
// }
//
// public void testAddToRI2() {
// long expected = TEST_TIME_NOW;
// expected = ISOChronology.getInstance().years().add(expected, -2);
// expected = ISOChronology.getInstance().months().add(expected, -4);
// expected = ISOChronology.getInstance().weeks().add(expected, -6);
// expected = ISOChronology.getInstance().days().add(expected, -8);
// expected = ISOChronology.getInstance().hours().add(expected, -10);
// expected = ISOChronology.getInstance().minutes().add(expected, -12);
// expected = ISOChronology.getInstance().seconds().add(expected, -14);
// expected = ISOChronology.getInstance().millis().add(expected, -16);
//
// Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8, PeriodType.standard());
// DateTime added = test.addTo(new Instant(), -2); // Instant has no time zone, use default
// assertEquals(expected, added.getMillis());
// assertEquals(ISOChronology.getInstance(), added.getChronology());
// }
//
// public void testAddToRI3() {
// long expected = TEST_TIME_NOW;
// expected = ISOChronology.getInstanceUTC().years().add(expected, -2);
// expected = ISOChronology.getInstanceUTC().months().add(expected, -4);
// expected = ISOChronology.getInstanceUTC().weeks().add(expected, -6);
// expected = ISOChronology.getInstanceUTC().days().add(expected, -8);
// expected = ISOChronology.getInstanceUTC().hours().add(expected, -10);
// expected = ISOChronology.getInstanceUTC().minutes().add(expected, -12);
// expected = ISOChronology.getInstanceUTC().seconds().add(expected, -14);
// expected = ISOChronology.getInstanceUTC().millis().add(expected, -16);
//
// Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8, PeriodType.standard());
// DateTime added = test.addTo(new DateTime(ISOChronology.getInstanceUTC()), -2); // DateTime has UTC time zone
// assertEquals(expected, added.getMillis());
// assertEquals(ISOChronology.getInstanceUTC(), added.getChronology());
// }
//
// public void testAddToRI4() {
// long expected = TEST_TIME_NOW;
// expected = ISOChronology.getInstance(PARIS).years().add(expected, -2);
// expected = ISOChronology.getInstance(PARIS).months().add(expected, -4);
// expected = ISOChronology.getInstance(PARIS).weeks().add(expected, -6);
// expected = ISOChronology.getInstance(PARIS).days().add(expected, -8);
// expected = ISOChronology.getInstance(PARIS).hours().add(expected, -10);
// expected = ISOChronology.getInstance(PARIS).minutes().add(expected, -12);
// expected = ISOChronology.getInstance(PARIS).seconds().add(expected, -14);
// expected = ISOChronology.getInstance(PARIS).millis().add(expected, -16);
//
// Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8, PeriodType.standard());
// DateTime added = test.addTo(new DateTime(PARIS), -2); // DateTime has PARIS time zone
// assertEquals(expected, added.getMillis());
// assertEquals(ISOChronology.getInstance(PARIS), added.getChronology());
// }
//
// public void testAddToRI5() {
// long expected = TEST_TIME_NOW;
// expected = ISOChronology.getInstance().years().add(expected, -2);
// expected = ISOChronology.getInstance().months().add(expected, -4);
// expected = ISOChronology.getInstance().weeks().add(expected, -6);
// expected = ISOChronology.getInstance().days().add(expected, -8);
// expected = ISOChronology.getInstance().hours().add(expected, -10);
// expected = ISOChronology.getInstance().minutes().add(expected, -12);
// expected = ISOChronology.getInstance().seconds().add(expected, -14);
// expected = ISOChronology.getInstance().millis().add(expected, -16);
//
// Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8, PeriodType.standard());
// DateTime added = test.addTo(null, -2); // null has no time zone, use default
// assertEquals(expected, added.getMillis());
// assertEquals(ISOChronology.getInstance(), added.getChronology());
// }
//
// //-----------------------------------------------------------------------
// public void testAddIntoRWI1() {
// long expected = TEST_TIME_NOW;
// expected = ISOChronology.getInstance().years().add(expected, 1);
// expected = ISOChronology.getInstance().months().add(expected, 2);
// expected = ISOChronology.getInstance().weeks().add(expected, 3);
// expected = ISOChronology.getInstance().days().add(expected, 4);
// expected = ISOChronology.getInstance().hours().add(expected, 5);
// expected = ISOChronology.getInstance().minutes().add(expected, 6);
// expected = ISOChronology.getInstance().seconds().add(expected, 7);
// expected = ISOChronology.getInstance().millis().add(expected, 8);
//
// Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8);
// MutableDateTime mdt = new MutableDateTime();
// test.addInto(mdt, 1);
// assertEquals(expected, mdt.getMillis());
// }
//
// public void testAddIntoRWI2() {
// long expected = TEST_TIME_NOW;
// expected = ISOChronology.getInstance().years().add(expected, -2);
// expected = ISOChronology.getInstance().months().add(expected, -4);
// expected = ISOChronology.getInstance().weeks().add(expected, -6);
// expected = ISOChronology.getInstance().days().add(expected, -8);
// expected = ISOChronology.getInstance().hours().add(expected, -10);
// expected = ISOChronology.getInstance().minutes().add(expected, -12);
// expected = ISOChronology.getInstance().seconds().add(expected, -14);
// expected = ISOChronology.getInstance().millis().add(expected, -16);
//
// Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8, PeriodType.standard());
// MutableDateTime mdt = new MutableDateTime();
// test.addInto(mdt, -2); // MutableDateTime has a chronology, use it
// assertEquals(expected, mdt.getMillis());
// }
//
// public void testAddIntoRWI3() {
// Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8);
// try {
// test.addInto(null, 1);
// fail();
// } catch (IllegalArgumentException ex) {}
// }
//-----------------------------------------------------------------------
public void testToString() {
Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8);
assertEquals("P1Y2M3W4DT5H6M7.008S", test.toString());
test = new Period(0, 0, 0, 0, 0, 0, 0, 0);
assertEquals("PT0S", test.toString());
test = new Period(12345L);
assertEquals("PT12.345S", test.toString());
}
//-----------------------------------------------------------------------
public void testToString_PeriodFormatter() {
Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8);
assertEquals("1 year, 2 months, 3 weeks, 4 days, 5 hours, 6 minutes, 7 seconds and 8 milliseconds", test.toString(PeriodFormat.getDefault()));
test = new Period(0, 0, 0, 0, 0, 0, 0, 0);
assertEquals("0 milliseconds", test.toString(PeriodFormat.getDefault()));
}
public void testToString_nullPeriodFormatter() {
Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8);
assertEquals("P1Y2M3W4DT5H6M7.008S", test.toString((PeriodFormatter) null));
}
//-----------------------------------------------------------------------
public void testToPeriod() {
Period test = new Period(123L);
Period result = test.toPeriod();
assertSame(test, result);
}
public void testToMutablePeriod() {
Period test = new Period(123L);
MutablePeriod result = test.toMutablePeriod();
assertEquals(test, result);
}
//-----------------------------------------------------------------------
// public void testToDurationMillisFrom() {
// Period test = new Period(123L);
// assertEquals(123L, test.toDurationMillisFrom(0L, null));
// }
public void testToDurationFrom() {
Period test = new Period(123L);
assertEquals(new Duration(123L), test.toDurationFrom(new Instant(0L)));
}
public void testToDurationTo() {
Period test = new Period(123L);
assertEquals(new Duration(123L), test.toDurationTo(new Instant(123L)));
}
//-----------------------------------------------------------------------
public void testWithPeriodType1() {
Period test = new Period(123L);
Period result = test.withPeriodType(PeriodType.standard());
assertSame(test, result);
}
public void testWithPeriodType2() {
Period test = new Period(3123L);
Period result = test.withPeriodType(PeriodType.dayTime());
assertEquals(3, result.getSeconds());
assertEquals(123, result.getMillis());
assertEquals(PeriodType.dayTime(), result.getPeriodType());
}
public void testWithPeriodType3() {
Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8, PeriodType.standard());
try {
test.withPeriodType(PeriodType.dayTime());
fail();
} catch (IllegalArgumentException ex) {}
}
public void testWithPeriodType4() {
Period test = new Period(3123L);
Period result = test.withPeriodType(null);
assertEquals(3, result.getSeconds());
assertEquals(123, result.getMillis());
assertEquals(PeriodType.standard(), result.getPeriodType());
}
public void testWithPeriodType5() {
Period test = new Period(1, 2, 0, 4, 5, 6, 7, 8, PeriodType.standard());
Period result = test.withPeriodType(PeriodType.yearMonthDayTime());
assertEquals(PeriodType.yearMonthDayTime(), result.getPeriodType());
assertEquals(1, result.getYears());
assertEquals(2, result.getMonths());
assertEquals(0, result.getWeeks());
assertEquals(4, result.getDays());
assertEquals(5, result.getHours());
assertEquals(6, result.getMinutes());
assertEquals(7, result.getSeconds());
assertEquals(8, result.getMillis());
}
//-----------------------------------------------------------------------
public void testWithFields1() {
Period test1 = new Period(1, 2, 3, 4, 5, 6, 7, 8);
Period test2 = new Period(0, 0, 0, 0, 0, 0, 0, 9, PeriodType.millis());
Period result = test1.withFields(test2);
assertEquals(new Period(1, 2, 3, 4, 5, 6, 7, 8), test1);
assertEquals(new Period(0, 0, 0, 0, 0, 0, 0, 9, PeriodType.millis()), test2);
assertEquals(new Period(1, 2, 3, 4, 5, 6, 7, 9), result);
}
public void testWithFields2() {
Period test1 = new Period(1, 2, 3, 4, 5, 6, 7, 8);
Period test2 = null;
Period result = test1.withFields(test2);
assertEquals(new Period(1, 2, 3, 4, 5, 6, 7, 8), test1);
assertSame(test1, result);
}
public void testWithFields3() {
Period test1 = new Period(0, 0, 0, 0, 0, 0, 0, 9, PeriodType.millis());
Period test2 = new Period(1, 2, 3, 4, 5, 6, 7, 8);
try {
test1.withFields(test2);
fail();
} catch (IllegalArgumentException ex) {}
assertEquals(new Period(0, 0, 0, 0, 0, 0, 0, 9, PeriodType.millis()), test1);
assertEquals(new Period(1, 2, 3, 4, 5, 6, 7, 8), test2);
}
//-----------------------------------------------------------------------
public void testWithField1() {
Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8);
Period result = test.withField(DurationFieldType.years(), 6);
assertEquals(new Period(1, 2, 3, 4, 5, 6, 7, 8), test);
assertEquals(new Period(6, 2, 3, 4, 5, 6, 7, 8), result);
}
public void testWithField2() {
Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8);
try {
test.withField(null, 6);
fail();
} catch (IllegalArgumentException ex) {}
}
public void testWithField3() {
Period test = new Period(0, 0, 0, 0, 5, 6, 7, 8, PeriodType.time());
try {
test.withField(DurationFieldType.years(), 6);
fail();
} catch (IllegalArgumentException ex) {}
}
public void testWithField4() {
Period test = new Period(0, 0, 0, 0, 5, 6, 7, 8, PeriodType.time());
Period result = test.withField(DurationFieldType.years(), 0);
assertEquals(test, result);
}
//-----------------------------------------------------------------------
public void testWithFieldAdded1() {
Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8);
Period result = test.withFieldAdded(DurationFieldType.years(), 6);
assertEquals(new Period(1, 2, 3, 4, 5, 6, 7, 8), test);
assertEquals(new Period(7, 2, 3, 4, 5, 6, 7, 8), result);
}
public void testWithFieldAdded2() {
Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8);
try {
test.withFieldAdded(null, 0);
fail();
} catch (IllegalArgumentException ex) {}
}
public void testWithFieldAdded3() {
Period test = new Period(0, 0, 0, 0, 5, 6, 7, 8, PeriodType.time());
try {
test.withFieldAdded(DurationFieldType.years(), 6);
fail();
} catch (IllegalArgumentException ex) {}
}
public void testWithFieldAdded4() {
Period test = new Period(0, 0, 0, 0, 5, 6, 7, 8, PeriodType.time());
Period result = test.withFieldAdded(DurationFieldType.years(), 0);
assertEquals(test, result);
}
//-----------------------------------------------------------------------
public void testPeriodStatics() {
Period test;
test = Period.years(1);
assertEquals(test, new Period(1, 0, 0, 0, 0, 0, 0, 0, PeriodType.standard()));
test = Period.months(1);
assertEquals(test, new Period(0, 1, 0, 0, 0, 0, 0, 0, PeriodType.standard()));
test = Period.weeks(1);
assertEquals(test, new Period(0, 0, 1, 0, 0, 0, 0, 0, PeriodType.standard()));
test = Period.days(1);
assertEquals(test, new Period(0, 0, 0, 1, 0, 0, 0, 0, PeriodType.standard()));
test = Period.hours(1);
assertEquals(test, new Period(0, 0, 0, 0, 1, 0, 0, 0, PeriodType.standard()));
test = Period.minutes(1);
assertEquals(test, new Period(0, 0, 0, 0, 0, 1, 0, 0, PeriodType.standard()));
test = Period.seconds(1);
assertEquals(test, new Period(0, 0, 0, 0, 0, 0, 1, 0, PeriodType.standard()));
test = Period.millis(1);
assertEquals(test, new Period(0, 0, 0, 0, 0, 0, 0, 1, PeriodType.standard()));
}
//-----------------------------------------------------------------------
public void testWith() {
Period test;
test = Period.years(5).withYears(1);
assertEquals(test, new Period(1, 0, 0, 0, 0, 0, 0, 0, PeriodType.standard()));
test = Period.months(5).withMonths(1);
assertEquals(test, new Period(0, 1, 0, 0, 0, 0, 0, 0, PeriodType.standard()));
test = Period.weeks(5).withWeeks(1);
assertEquals(test, new Period(0, 0, 1, 0, 0, 0, 0, 0, PeriodType.standard()));
test = Period.days(5).withDays(1);
assertEquals(test, new Period(0, 0, 0, 1, 0, 0, 0, 0, PeriodType.standard()));
test = Period.hours(5).withHours(1);
assertEquals(test, new Period(0, 0, 0, 0, 1, 0, 0, 0, PeriodType.standard()));
test = Period.minutes(5).withMinutes(1);
assertEquals(test, new Period(0, 0, 0, 0, 0, 1, 0, 0, PeriodType.standard()));
test = Period.seconds(5).withSeconds(1);
assertEquals(test, new Period(0, 0, 0, 0, 0, 0, 1, 0, PeriodType.standard()));
test = Period.millis(5).withMillis(1);
assertEquals(test, new Period(0, 0, 0, 0, 0, 0, 0, 1, PeriodType.standard()));
test = new Period(0L, PeriodType.millis());
try {
test.withYears(1);
fail();
} catch (UnsupportedOperationException ex) {}
}
//-----------------------------------------------------------------------
public void testPlus() {
Period base = new Period(1, 2, 3, 4, 5, 6, 7, 8);
Period baseDaysOnly = new Period(0, 0, 0, 10, 0, 0, 0, 0, PeriodType.days());
Period test = base.plus((ReadablePeriod) null);
assertSame(base, test);
test = base.plus(Period.years(10));
assertEquals(11, test.getYears());
assertEquals(2, test.getMonths());
assertEquals(3, test.getWeeks());
assertEquals(4, test.getDays());
assertEquals(5, test.getHours());
assertEquals(6, test.getMinutes());
assertEquals(7, test.getSeconds());
assertEquals(8, test.getMillis());
test = base.plus(Years.years(10));
assertEquals(11, test.getYears());
assertEquals(2, test.getMonths());
assertEquals(3, test.getWeeks());
assertEquals(4, test.getDays());
assertEquals(5, test.getHours());
assertEquals(6, test.getMinutes());
assertEquals(7, test.getSeconds());
assertEquals(8, test.getMillis());
test = base.plus(Period.days(10));
assertEquals(1, test.getYears());
assertEquals(2, test.getMonths());
assertEquals(3, test.getWeeks());
assertEquals(14, test.getDays());
assertEquals(5, test.getHours());
assertEquals(6, test.getMinutes());
assertEquals(7, test.getSeconds());
assertEquals(8, test.getMillis());
test = baseDaysOnly.plus(Period.years(0));
assertEquals(0, test.getYears());
assertEquals(0, test.getMonths());
assertEquals(0, test.getWeeks());
assertEquals(10, test.getDays());
assertEquals(0, test.getHours());
assertEquals(0, test.getMinutes());
assertEquals(0, test.getSeconds());
assertEquals(0, test.getMillis());
test = baseDaysOnly.plus(baseDaysOnly);
assertEquals(0, test.getYears());
assertEquals(0, test.getMonths());
assertEquals(0, test.getWeeks());
assertEquals(20, test.getDays());
assertEquals(0, test.getHours());
assertEquals(0, test.getMinutes());
assertEquals(0, test.getSeconds());
assertEquals(0, test.getMillis());
try {
baseDaysOnly.plus(Period.years(1));
fail();
} catch (UnsupportedOperationException ex) {}
try {
Period.days(Integer.MAX_VALUE).plus(Period.days(1));
fail();
} catch (ArithmeticException ex) {}
try {
Period.days(Integer.MIN_VALUE).plus(Period.days(-1));
fail();
} catch (ArithmeticException ex) {}
}
//-----------------------------------------------------------------------
public void testMinus() {
Period base = new Period(1, 2, 3, 4, 5, 6, 7, 8);
Period baseDaysOnly = new Period(0, 0, 0, 10, 0, 0, 0, 0, PeriodType.days());
Period test = base.minus((ReadablePeriod) null);
assertSame(base, test);
test = base.minus(Period.years(10));
assertEquals(-9, test.getYears());
assertEquals(2, test.getMonths());
assertEquals(3, test.getWeeks());
assertEquals(4, test.getDays());
assertEquals(5, test.getHours());
assertEquals(6, test.getMinutes());
assertEquals(7, test.getSeconds());
assertEquals(8, test.getMillis());
test = base.minus(Years.years(10));
assertEquals(-9, test.getYears());
assertEquals(2, test.getMonths());
assertEquals(3, test.getWeeks());
assertEquals(4, test.getDays());
assertEquals(5, test.getHours());
assertEquals(6, test.getMinutes());
assertEquals(7, test.getSeconds());
assertEquals(8, test.getMillis());
test = base.minus(Period.days(10));
assertEquals(1, test.getYears());
assertEquals(2, test.getMonths());
assertEquals(3, test.getWeeks());
assertEquals(-6, test.getDays());
assertEquals(5, test.getHours());
assertEquals(6, test.getMinutes());
assertEquals(7, test.getSeconds());
assertEquals(8, test.getMillis());
test = baseDaysOnly.minus(Period.years(0));
assertEquals(0, test.getYears());
assertEquals(0, test.getMonths());
assertEquals(0, test.getWeeks());
assertEquals(10, test.getDays());
assertEquals(0, test.getHours());
assertEquals(0, test.getMinutes());
assertEquals(0, test.getSeconds());
assertEquals(0, test.getMillis());
test = baseDaysOnly.minus(baseDaysOnly);
assertEquals(0, test.getYears());
assertEquals(0, test.getMonths());
assertEquals(0, test.getWeeks());
assertEquals(0, test.getDays());
assertEquals(0, test.getHours());
assertEquals(0, test.getMinutes());
assertEquals(0, test.getSeconds());
assertEquals(0, test.getMillis());
try {
baseDaysOnly.minus(Period.years(1));
fail();
} catch (UnsupportedOperationException ex) {}
try {
Period.days(Integer.MAX_VALUE).minus(Period.days(-1));
fail();
} catch (ArithmeticException ex) {}
try {
Period.days(Integer.MIN_VALUE).minus(Period.days(1));
fail();
} catch (ArithmeticException ex) {}
}
//-----------------------------------------------------------------------
public void testPlusFields() {
Period test;
test = Period.years(1).plusYears(1);
assertEquals(new Period(2, 0, 0, 0, 0, 0, 0, 0, PeriodType.standard()), test);
test = Period.months(1).plusMonths(1);
assertEquals(new Period(0, 2, 0, 0, 0, 0, 0, 0, PeriodType.standard()), test);
test = Period.weeks(1).plusWeeks(1);
assertEquals(new Period(0, 0, 2, 0, 0, 0, 0, 0, PeriodType.standard()), test);
test = Period.days(1).plusDays(1);
assertEquals(new Period(0, 0, 0, 2, 0, 0, 0, 0, PeriodType.standard()), test);
test = Period.hours(1).plusHours(1);
assertEquals(new Period(0, 0, 0, 0, 2, 0, 0, 0, PeriodType.standard()), test);
test = Period.minutes(1).plusMinutes(1);
assertEquals(new Period(0, 0, 0, 0, 0, 2, 0, 0, PeriodType.standard()), test);
test = Period.seconds(1).plusSeconds(1);
assertEquals(new Period(0, 0, 0, 0, 0, 0, 2, 0, PeriodType.standard()), test);
test = Period.millis(1).plusMillis(1);
assertEquals(new Period(0, 0, 0, 0, 0, 0, 0, 2, PeriodType.standard()), test);
test = new Period(0L, PeriodType.millis());
try {
test.plusYears(1);
fail();
} catch (UnsupportedOperationException ex) {}
}
public void testPlusFieldsZero() {
Period test, result;
test = Period.years(1);
result = test.plusYears(0);
assertSame(test, result);
test = Period.months(1);
result = test.plusMonths(0);
assertSame(test, result);
test = Period.weeks(1);
result = test.plusWeeks(0);
assertSame(test, result);
test = Period.days(1);
result = test.plusDays(0);
assertSame(test, result);
test = Period.hours(1);
result = test.plusHours(0);
assertSame(test, result);
test = Period.minutes(1);
result = test.plusMinutes(0);
assertSame(test, result);
test = Period.seconds(1);
result = test.plusSeconds(0);
assertSame(test, result);
test = Period.millis(1);
result = test.plusMillis(0);
assertSame(test, result);
}
public void testMinusFields() {
Period test;
test = Period.years(3).minusYears(1);
assertEquals(new Period(2, 0, 0, 0, 0, 0, 0, 0, PeriodType.standard()), test);
test = Period.months(3).minusMonths(1);
assertEquals(new Period(0, 2, 0, 0, 0, 0, 0, 0, PeriodType.standard()), test);
test = Period.weeks(3).minusWeeks(1);
assertEquals(new Period(0, 0, 2, 0, 0, 0, 0, 0, PeriodType.standard()), test);
test = Period.days(3).minusDays(1);
assertEquals(new Period(0, 0, 0, 2, 0, 0, 0, 0, PeriodType.standard()), test);
test = Period.hours(3).minusHours(1);
assertEquals(new Period(0, 0, 0, 0, 2, 0, 0, 0, PeriodType.standard()), test);
test = Period.minutes(3).minusMinutes(1);
assertEquals(new Period(0, 0, 0, 0, 0, 2, 0, 0, PeriodType.standard()), test);
test = Period.seconds(3).minusSeconds(1);
assertEquals(new Period(0, 0, 0, 0, 0, 0, 2, 0, PeriodType.standard()), test);
test = Period.millis(3).minusMillis(1);
assertEquals(new Period(0, 0, 0, 0, 0, 0, 0, 2, PeriodType.standard()), test);
test = new Period(0L, PeriodType.millis());
try {
test.minusYears(1);
fail();
} catch (UnsupportedOperationException ex) {}
}
//-----------------------------------------------------------------------
public void testMultipliedBy() {
Period base = new Period(1, 2, 3, 4, 5, 6, 7, 8);
Period test = base.multipliedBy(1);
assertSame(base, test);
test = base.multipliedBy(0);
assertEquals(Period.ZERO, test);
test = base.multipliedBy(2);
assertEquals(2, test.getYears());
assertEquals(4, test.getMonths());
assertEquals(6, test.getWeeks());
assertEquals(8, test.getDays());
assertEquals(10, test.getHours());
assertEquals(12, test.getMinutes());
assertEquals(14, test.getSeconds());
assertEquals(16, test.getMillis());
test = base.multipliedBy(3);
assertEquals(3, test.getYears());
assertEquals(6, test.getMonths());
assertEquals(9, test.getWeeks());
assertEquals(12, test.getDays());
assertEquals(15, test.getHours());
assertEquals(18, test.getMinutes());
assertEquals(21, test.getSeconds());
assertEquals(24, test.getMillis());
test = base.multipliedBy(-4);
assertEquals(-4, test.getYears());
assertEquals(-8, test.getMonths());
assertEquals(-12, test.getWeeks());
assertEquals(-16, test.getDays());
assertEquals(-20, test.getHours());
assertEquals(-24, test.getMinutes());
assertEquals(-28, test.getSeconds());
assertEquals(-32, test.getMillis());
try {
Period.days(Integer.MAX_VALUE).multipliedBy(2);
fail();
} catch (ArithmeticException ex) {}
try {
Period.days(Integer.MIN_VALUE).multipliedBy(2);
fail();
} catch (ArithmeticException ex) {}
}
//-----------------------------------------------------------------------
public void testNegated() {
Period base = new Period(1, 2, 3, 4, 5, 6, 7, 8);
Period test = Period.ZERO.negated();
assertEquals(Period.ZERO, test);
test = base.negated();
assertEquals(-1, test.getYears());
assertEquals(-2, test.getMonths());
assertEquals(-3, test.getWeeks());
assertEquals(-4, test.getDays());
assertEquals(-5, test.getHours());
assertEquals(-6, test.getMinutes());
assertEquals(-7, test.getSeconds());
assertEquals(-8, test.getMillis());
test = Period.days(Integer.MAX_VALUE).negated();
assertEquals(-Integer.MAX_VALUE, test.getDays());
try {
Period.days(Integer.MIN_VALUE).negated();
fail();
} catch (ArithmeticException ex) {}
}
//-----------------------------------------------------------------------
public void testToStandardWeeks() {
Period test = new Period(0, 0, 3, 4, 5, 6, 7, 8);
assertEquals(3, test.toStandardWeeks().getWeeks());
test = new Period(0, 0, 3, 7, 0, 0, 0, 0);
assertEquals(4, test.toStandardWeeks().getWeeks());
test = new Period(0, 0, 0, 6, 23, 59, 59, 1000);
assertEquals(1, test.toStandardWeeks().getWeeks());
test = new Period(0, 0, Integer.MAX_VALUE, 0, 0, 0, 0, 0);
assertEquals(Integer.MAX_VALUE, test.toStandardWeeks().getWeeks());
test = new Period(0, 0, 0, Integer.MAX_VALUE, Integer.MAX_VALUE, Integer.MAX_VALUE, Integer.MAX_VALUE, Integer.MAX_VALUE);
long intMax = Integer.MAX_VALUE;
BigInteger expected = BigInteger.valueOf(intMax);
expected = expected.add(BigInteger.valueOf(intMax * DateTimeConstants.MILLIS_PER_SECOND));
expected = expected.add(BigInteger.valueOf(intMax * DateTimeConstants.MILLIS_PER_MINUTE));
expected = expected.add(BigInteger.valueOf(intMax * DateTimeConstants.MILLIS_PER_HOUR));
expected = expected.add(BigInteger.valueOf(intMax * DateTimeConstants.MILLIS_PER_DAY));
expected = expected.divide(BigInteger.valueOf(DateTimeConstants.MILLIS_PER_WEEK));
assertTrue(expected.compareTo(BigInteger.valueOf(Long.MAX_VALUE)) < 0);
assertEquals(expected.longValue(), test.toStandardWeeks().getWeeks());
test = new Period(0, 0, Integer.MAX_VALUE, 7, 0, 0, 0, 0);
try {
test.toStandardWeeks();
fail();
} catch (ArithmeticException ex) {}
}
public void testToStandardWeeks_years() {
Period test = Period.years(1);
try {
test.toStandardWeeks();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.years(-1);
try {
test.toStandardWeeks();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.years(0);
assertEquals(0, test.toStandardWeeks().getWeeks());
}
public void testToStandardWeeks_months() {
Period test = Period.months(1);
try {
test.toStandardWeeks();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.months(-1);
try {
test.toStandardWeeks();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.months(0);
assertEquals(0, test.toStandardWeeks().getWeeks());
}
//-----------------------------------------------------------------------
public void testToStandardDays() {
Period test = new Period(0, 0, 0, 4, 5, 6, 7, 8);
assertEquals(4, test.toStandardDays().getDays());
test = new Period(0, 0, 1, 4, 0, 0, 0, 0);
assertEquals(11, test.toStandardDays().getDays());
test = new Period(0, 0, 0, 0, 23, 59, 59, 1000);
assertEquals(1, test.toStandardDays().getDays());
test = new Period(0, 0, 0, Integer.MAX_VALUE, 0, 0, 0, 0);
assertEquals(Integer.MAX_VALUE, test.toStandardDays().getDays());
test = new Period(0, 0, 0, 0, Integer.MAX_VALUE, Integer.MAX_VALUE, Integer.MAX_VALUE, Integer.MAX_VALUE);
long intMax = Integer.MAX_VALUE;
BigInteger expected = BigInteger.valueOf(intMax);
expected = expected.add(BigInteger.valueOf(intMax * DateTimeConstants.MILLIS_PER_SECOND));
expected = expected.add(BigInteger.valueOf(intMax * DateTimeConstants.MILLIS_PER_MINUTE));
expected = expected.add(BigInteger.valueOf(intMax * DateTimeConstants.MILLIS_PER_HOUR));
expected = expected.divide(BigInteger.valueOf(DateTimeConstants.MILLIS_PER_DAY));
assertTrue(expected.compareTo(BigInteger.valueOf(Long.MAX_VALUE)) < 0);
assertEquals(expected.longValue(), test.toStandardDays().getDays());
test = new Period(0, 0, 0, Integer.MAX_VALUE, 24, 0, 0, 0);
try {
test.toStandardDays();
fail();
} catch (ArithmeticException ex) {}
}
public void testToStandardDays_years() {
Period test = Period.years(1);
try {
test.toStandardDays();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.years(-1);
try {
test.toStandardDays();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.years(0);
assertEquals(0, test.toStandardDays().getDays());
}
public void testToStandardDays_months() {
Period test = Period.months(1);
try {
test.toStandardDays();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.months(-1);
try {
test.toStandardDays();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.months(0);
assertEquals(0, test.toStandardDays().getDays());
}
//-----------------------------------------------------------------------
public void testToStandardHours() {
Period test = new Period(0, 0, 0, 0, 5, 6, 7, 8);
assertEquals(5, test.toStandardHours().getHours());
test = new Period(0, 0, 0, 1, 5, 0, 0, 0);
assertEquals(29, test.toStandardHours().getHours());
test = new Period(0, 0, 0, 0, 0, 59, 59, 1000);
assertEquals(1, test.toStandardHours().getHours());
test = new Period(0, 0, 0, 0, Integer.MAX_VALUE, 0, 0, 0);
assertEquals(Integer.MAX_VALUE, test.toStandardHours().getHours());
test = new Period(0, 0, 0, 0, 0, Integer.MAX_VALUE, Integer.MAX_VALUE, Integer.MAX_VALUE);
long intMax = Integer.MAX_VALUE;
BigInteger expected = BigInteger.valueOf(intMax);
expected = expected.add(BigInteger.valueOf(intMax * DateTimeConstants.MILLIS_PER_SECOND));
expected = expected.add(BigInteger.valueOf(intMax * DateTimeConstants.MILLIS_PER_MINUTE));
expected = expected.divide(BigInteger.valueOf(DateTimeConstants.MILLIS_PER_HOUR));
assertTrue(expected.compareTo(BigInteger.valueOf(Long.MAX_VALUE)) < 0);
assertEquals(expected.longValue(), test.toStandardHours().getHours());
test = new Period(0, 0, 0, 0, Integer.MAX_VALUE, 60, 0, 0);
try {
test.toStandardHours();
fail();
} catch (ArithmeticException ex) {}
}
public void testToStandardHours_years() {
Period test = Period.years(1);
try {
test.toStandardHours();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.years(-1);
try {
test.toStandardHours();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.years(0);
assertEquals(0, test.toStandardHours().getHours());
}
public void testToStandardHours_months() {
Period test = Period.months(1);
try {
test.toStandardHours();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.months(-1);
try {
test.toStandardHours();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.months(0);
assertEquals(0, test.toStandardHours().getHours());
}
//-----------------------------------------------------------------------
public void testToStandardMinutes() {
Period test = new Period(0, 0, 0, 0, 0, 6, 7, 8);
assertEquals(6, test.toStandardMinutes().getMinutes());
test = new Period(0, 0, 0, 0, 1, 6, 0, 0);
assertEquals(66, test.toStandardMinutes().getMinutes());
test = new Period(0, 0, 0, 0, 0, 0, 59, 1000);
assertEquals(1, test.toStandardMinutes().getMinutes());
test = new Period(0, 0, 0, 0, 0, Integer.MAX_VALUE, 0, 0);
assertEquals(Integer.MAX_VALUE, test.toStandardMinutes().getMinutes());
test = new Period(0, 0, 0, 0, 0, 0, Integer.MAX_VALUE, Integer.MAX_VALUE);
long intMax = Integer.MAX_VALUE;
BigInteger expected = BigInteger.valueOf(intMax);
expected = expected.add(BigInteger.valueOf(intMax * DateTimeConstants.MILLIS_PER_SECOND));
expected = expected.divide(BigInteger.valueOf(DateTimeConstants.MILLIS_PER_MINUTE));
assertTrue(expected.compareTo(BigInteger.valueOf(Long.MAX_VALUE)) < 0);
assertEquals(expected.longValue(), test.toStandardMinutes().getMinutes());
test = new Period(0, 0, 0, 0, 0, Integer.MAX_VALUE, 60, 0);
try {
test.toStandardMinutes();
fail();
} catch (ArithmeticException ex) {}
}
public void testToStandardMinutes_years() {
Period test = Period.years(1);
try {
test.toStandardMinutes();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.years(-1);
try {
test.toStandardMinutes();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.years(0);
assertEquals(0, test.toStandardMinutes().getMinutes());
}
public void testToStandardMinutes_months() {
Period test = Period.months(1);
try {
test.toStandardMinutes();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.months(-1);
try {
test.toStandardMinutes();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.months(0);
assertEquals(0, test.toStandardMinutes().getMinutes());
}
//-----------------------------------------------------------------------
public void testToStandardSeconds() {
Period test = new Period(0, 0, 0, 0, 0, 0, 7, 8);
assertEquals(7, test.toStandardSeconds().getSeconds());
test = new Period(0, 0, 0, 0, 0, 1, 3, 0);
assertEquals(63, test.toStandardSeconds().getSeconds());
test = new Period(0, 0, 0, 0, 0, 0, 0, 1000);
assertEquals(1, test.toStandardSeconds().getSeconds());
test = new Period(0, 0, 0, 0, 0, 0, Integer.MAX_VALUE, 0);
assertEquals(Integer.MAX_VALUE, test.toStandardSeconds().getSeconds());
test = new Period(0, 0, 0, 0, 0, 0, 20, Integer.MAX_VALUE);
long expected = 20;
expected += ((long) Integer.MAX_VALUE) / DateTimeConstants.MILLIS_PER_SECOND;
assertEquals(expected, test.toStandardSeconds().getSeconds());
test = new Period(0, 0, 0, 0, 0, 0, Integer.MAX_VALUE, 1000);
try {
test.toStandardSeconds();
fail();
} catch (ArithmeticException ex) {}
}
public void testToStandardSeconds_years() {
Period test = Period.years(1);
try {
test.toStandardSeconds();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.years(-1);
try {
test.toStandardSeconds();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.years(0);
assertEquals(0, test.toStandardSeconds().getSeconds());
}
public void testToStandardSeconds_months() {
Period test = Period.months(1);
try {
test.toStandardSeconds();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.months(-1);
try {
test.toStandardSeconds();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.months(0);
assertEquals(0, test.toStandardSeconds().getSeconds());
}
//-----------------------------------------------------------------------
public void testToStandardDuration() {
Period test = new Period(0, 0, 0, 0, 0, 0, 0, 8);
assertEquals(8, test.toStandardDuration().getMillis());
test = new Period(0, 0, 0, 0, 0, 0, 1, 20);
assertEquals(1020, test.toStandardDuration().getMillis());
test = new Period(0, 0, 0, 0, 0, 0, 0, Integer.MAX_VALUE);
assertEquals(Integer.MAX_VALUE, test.toStandardDuration().getMillis());
test = new Period(0, 0, 0, 0, 0, 10, 20, Integer.MAX_VALUE);
long expected = Integer.MAX_VALUE;
expected += 10L * ((long) DateTimeConstants.MILLIS_PER_MINUTE);
expected += 20L * ((long) DateTimeConstants.MILLIS_PER_SECOND);
assertEquals(expected, test.toStandardDuration().getMillis());
// proof that overflow does not occur
BigInteger intMax = BigInteger.valueOf(Integer.MAX_VALUE);
BigInteger exp = intMax;
exp = exp.add(intMax.multiply(BigInteger.valueOf(DateTimeConstants.MILLIS_PER_SECOND)));
exp = exp.add(intMax.multiply(BigInteger.valueOf(DateTimeConstants.MILLIS_PER_MINUTE)));
exp = exp.add(intMax.multiply(BigInteger.valueOf(DateTimeConstants.MILLIS_PER_HOUR)));
exp = exp.add(intMax.multiply(BigInteger.valueOf(DateTimeConstants.MILLIS_PER_DAY)));
exp = exp.add(intMax.multiply(BigInteger.valueOf(DateTimeConstants.MILLIS_PER_WEEK)));
assertTrue(exp.compareTo(BigInteger.valueOf(Long.MAX_VALUE)) < 0);
// test = new Period(0, 0, Integer.MAX_VALUE, Integer.MAX_VALUE, Integer.MAX_VALUE, Integer.MAX_VALUE, Integer.MAX_VALUE, Integer.MAX_VALUE);
// try {
// test.toStandardDuration();
// fail();
// } catch (ArithmeticException ex) {}
}
public void testToStandardDuration_years() {
Period test = Period.years(1);
try {
test.toStandardDuration();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.years(-1);
try {
test.toStandardDuration();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.years(0);
assertEquals(0, test.toStandardDuration().getMillis());
}
public void testToStandardDuration_months() {
Period test = Period.months(1);
try {
test.toStandardDuration();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.months(-1);
try {
test.toStandardDuration();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.months(0);
assertEquals(0, test.toStandardDuration().getMillis());
}
//-----------------------------------------------------------------------
public void testNormalizedStandard_yearMonth1() {
Period test = new Period(1, 15, 0, 0, 0, 0, 0, 0);
Period result = test.normalizedStandard();
assertEquals(new Period(1, 15, 0, 0, 0, 0, 0, 0), test);
assertEquals(new Period(2, 3, 0, 0, 0, 0, 0, 0), result);
}
public void testNormalizedStandard_yearMonth2() {
Period test = new Period(Integer.MAX_VALUE, 15, 0, 0, 0, 0, 0, 0);
try {
test.normalizedStandard();
fail();
} catch (ArithmeticException ex) {}
}
public void testNormalizedStandard_weekDay1() {
Period test = new Period(0, 0, 1, 12, 0, 0, 0, 0);
Period result = test.normalizedStandard();
assertEquals(new Period(0, 0, 1, 12, 0, 0, 0, 0), test);
assertEquals(new Period(0, 0, 2, 5, 0, 0, 0, 0), result);
}
public void testNormalizedStandard_weekDay2() {
Period test = new Period(0, 0, Integer.MAX_VALUE, 7, 0, 0, 0, 0);
try {
test.normalizedStandard();
fail();
} catch (ArithmeticException ex) {}
}
public void testNormalizedStandard_yearMonthWeekDay() {
Period test = new Period(1, 15, 1, 12, 0, 0, 0, 0);
Period result = test.normalizedStandard();
assertEquals(new Period(1, 15, 1, 12, 0, 0, 0, 0), test);
assertEquals(new Period(2, 3, 2, 5, 0, 0, 0, 0), result);
}
public void testNormalizedStandard_yearMonthDay() {
Period test = new Period(1, 15, 0, 36, 0, 0, 0, 0);
Period result = test.normalizedStandard();
assertEquals(new Period(1, 15, 0, 36, 0, 0, 0, 0), test);
assertEquals(new Period(2, 3, 5, 1, 0, 0, 0, 0), result);
}
public void testNormalizedStandard_negative() {
Period test = new Period(0, 0, 0, 0, 2, -10, 0, 0);
Period result = test.normalizedStandard();
assertEquals(new Period(0, 0, 0, 0, 2, -10, 0, 0), test);
assertEquals(new Period(0, 0, 0, 0, 1, 50, 0, 0), result);
}
public void testNormalizedStandard_fullNegative() {
Period test = new Period(0, 0, 0, 0, 1, -70, 0, 0);
Period result = test.normalizedStandard();
assertEquals(new Period(0, 0, 0, 0, 1, -70, 0, 0), test);
assertEquals(new Period(0, 0, 0, 0, 0, -10, 0, 0), result);
}
//-----------------------------------------------------------------------
public void testNormalizedStandard_periodType_yearMonth1() {
Period test = new Period(1, 15, 0, 0, 0, 0, 0, 0);
Period result = test.normalizedStandard((PeriodType) null);
assertEquals(new Period(1, 15, 0, 0, 0, 0, 0, 0), test);
assertEquals(new Period(2, 3, 0, 0, 0, 0, 0, 0), result);
}
public void testNormalizedStandard_periodType_yearMonth2() {
Period test = new Period(Integer.MAX_VALUE, 15, 0, 0, 0, 0, 0, 0);
try {
test.normalizedStandard((PeriodType) null);
fail();
} catch (ArithmeticException ex) {}
}
public void testNormalizedStandard_periodType_yearMonth3() {
Period test = new Period(1, 15, 3, 4, 0, 0, 0, 0);
try {
test.normalizedStandard(PeriodType.dayTime());
fail();
} catch (UnsupportedOperationException ex) {}
}
public void testNormalizedStandard_periodType_weekDay1() {
Period test = new Period(0, 0, 1, 12, 0, 0, 0, 0);
Period result = test.normalizedStandard((PeriodType) null);
assertEquals(new Period(0, 0, 1, 12, 0, 0, 0, 0), test);
assertEquals(new Period(0, 0, 2, 5, 0, 0, 0, 0), result);
}
public void testNormalizedStandard_periodType_weekDay2() {
Period test = new Period(0, 0, Integer.MAX_VALUE, 7, 0, 0, 0, 0);
try {
test.normalizedStandard((PeriodType) null);
fail();
} catch (ArithmeticException ex) {}
}
public void testNormalizedStandard_periodType_weekDay3() {
Period test = new Period(0, 0, 1, 12, 0, 0, 0, 0);
Period result = test.normalizedStandard(PeriodType.dayTime());
assertEquals(new Period(0, 0, 1, 12, 0, 0, 0, 0), test);
assertEquals(new Period(0, 0, 0, 19, 0, 0, 0, 0, PeriodType.dayTime()), result);
}
public void testNormalizedStandard_periodType_yearMonthWeekDay() {
Period test = new Period(1, 15, 1, 12, 0, 0, 0, 0);
Period result = test.normalizedStandard(PeriodType.yearMonthDayTime());
assertEquals(new Period(1, 15, 1, 12, 0, 0, 0, 0), test);
assertEquals(new Period(2, 3, 0, 19, 0, 0, 0, 0, PeriodType.yearMonthDayTime()), result);
}
public void testNormalizedStandard_periodType_yearMonthDay() {
Period test = new Period(1, 15, 0, 36, 27, 0, 0, 0);
Period result = test.normalizedStandard(PeriodType.yearMonthDayTime());
assertEquals(new Period(1, 15, 0, 36, 27, 0, 0, 0), test);
assertEquals(new Period(2, 3, 0, 37, 3, 0, 0, 0, PeriodType.yearMonthDayTime()), result);
}
public void testNormalizedStandard_periodType_months1() {
Period test = new Period(1, 15, 0, 0, 0, 0, 0, 0);
Period result = test.normalizedStandard(PeriodType.months());
assertEquals(new Period(1, 15, 0, 0, 0, 0, 0, 0), test);
assertEquals(new Period(0, 27, 0, 0, 0, 0, 0, 0, PeriodType.months()), result);
}
public void testNormalizedStandard_periodType_months2() {
Period test = new Period(-2, 15, 0, 0, 0, 0, 0, 0);
Period result = test.normalizedStandard(PeriodType.months());
assertEquals(new Period(-2, 15, 0, 0, 0, 0, 0, 0), test);
assertEquals(new Period(0, -9, 0, 0, 0, 0, 0, 0, PeriodType.months()), result);
}
public void testNormalizedStandard_periodType_months3() {
Period test = new Period(0, 4, 0, 0, 0, 0, 0, 0);
Period result = test.normalizedStandard(PeriodType.months());
assertEquals(new Period(0, 4, 0, 0, 0, 0, 0, 0), test);
assertEquals(new Period(0, 4, 0, 0, 0, 0, 0, 0, PeriodType.months()), result);
}
public void testNormalizedStandard_periodType_years() {
Period test = new Period(1, 15, 0, 0, 0, 0, 0, 0);
try {
test.normalizedStandard(PeriodType.years());
fail();
} catch (UnsupportedOperationException ex) {
// expected
}
}
public void testNormalizedStandard_periodType_monthsWeeks() {
PeriodType type = PeriodType.forFields(new DurationFieldType[]{
DurationFieldType.months(),
DurationFieldType.weeks(),
DurationFieldType.days()});
Period test = new Period(2, 4, 6, 0, 0, 0, 0, 0);
Period result = test.normalizedStandard(type);
assertEquals(new Period(2, 4, 6, 0, 0, 0, 0, 0), test);
assertEquals(new Period(0, 28, 6, 0, 0, 0, 0, 0, type), result);
}
} | // You are a professional Java test case writer, please create a test case named `testNormalizedStandard_periodType_months1` for the issue `Time-79`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Time-79
//
// ## Issue-Title:
// none standard PeriodType without year throws exception
//
// ## Issue-Description:
// Hi.
//
//
// I tried to get a Period only for months and weeks with following code:
//
//
//
// ```
// Period p = new Period(new DateTime(startDate.getTime()), new DateTime(endDate.getTime()), PeriodType.forFields(new DurationFieldType[]{DurationFieldType.months(), DurationFieldType.weeks()})).normalizedStandard(PeriodType.forFields(new DurationFieldType[]{DurationFieldType.months(), DurationFieldType.weeks()}));
// return p.getMonths();
// ```
//
// This throws following exception:
//
//
//
// ```
// 10-17 14:35:50.999: E/AndroidRuntime(1350): java.lang.UnsupportedOperationException: Field is not supported
// 10-17 14:35:50.999: E/AndroidRuntime(1350): at org.joda.time.PeriodType.setIndexedField(PeriodType.java:690)
// 10-17 14:35:50.999: E/AndroidRuntime(1350): at org.joda.time.Period.withYears(Period.java:896) 10-17
// 14:35:50.999: E/AndroidRuntime(1350): at org.joda.time.Period.normalizedStandard(Period.java:1630)
//
// ```
//
// Even removing the year component with .withYearsRemoved() throws the same exception:
//
//
// this works:
//
//
//
// ```
// Period p = new Period(new DateTime(startDate.getTime()), new DateTime(endDate.getTime()), PeriodType.standard()).normalizedStandard(PeriodType.standard());
// return p.getMonths();
// ```
//
// this fails:
//
//
//
// ```
// Period p = new Period(new DateTime(startDate.getTime()), new DateTime(endDate.getTime()), PeriodType.standard().withYearsRemoved()).normalizedStandard(PeriodType.standard().withYearsRemoved());
// return p.getMonths();
// ```
//
//
//
public void testNormalizedStandard_periodType_months1() {
| 1,524 | 5 | 1,519 | src/test/java/org/joda/time/TestPeriod_Basics.java | src/test/java | ```markdown
## Issue-ID: Time-79
## Issue-Title:
none standard PeriodType without year throws exception
## Issue-Description:
Hi.
I tried to get a Period only for months and weeks with following code:
```
Period p = new Period(new DateTime(startDate.getTime()), new DateTime(endDate.getTime()), PeriodType.forFields(new DurationFieldType[]{DurationFieldType.months(), DurationFieldType.weeks()})).normalizedStandard(PeriodType.forFields(new DurationFieldType[]{DurationFieldType.months(), DurationFieldType.weeks()}));
return p.getMonths();
```
This throws following exception:
```
10-17 14:35:50.999: E/AndroidRuntime(1350): java.lang.UnsupportedOperationException: Field is not supported
10-17 14:35:50.999: E/AndroidRuntime(1350): at org.joda.time.PeriodType.setIndexedField(PeriodType.java:690)
10-17 14:35:50.999: E/AndroidRuntime(1350): at org.joda.time.Period.withYears(Period.java:896) 10-17
14:35:50.999: E/AndroidRuntime(1350): at org.joda.time.Period.normalizedStandard(Period.java:1630)
```
Even removing the year component with .withYearsRemoved() throws the same exception:
this works:
```
Period p = new Period(new DateTime(startDate.getTime()), new DateTime(endDate.getTime()), PeriodType.standard()).normalizedStandard(PeriodType.standard());
return p.getMonths();
```
this fails:
```
Period p = new Period(new DateTime(startDate.getTime()), new DateTime(endDate.getTime()), PeriodType.standard().withYearsRemoved()).normalizedStandard(PeriodType.standard().withYearsRemoved());
return p.getMonths();
```
```
You are a professional Java test case writer, please create a test case named `testNormalizedStandard_periodType_months1` for the issue `Time-79`, utilizing the provided issue report information and the following function signature.
```java
public void testNormalizedStandard_periodType_months1() {
```
| 1,519 | [
"org.joda.time.Period"
] | 8b42e2cdd846820c376c547a8c58660ea991a11910b0ed15e2ce5d553cdb1d4b | public void testNormalizedStandard_periodType_months1() | // You are a professional Java test case writer, please create a test case named `testNormalizedStandard_periodType_months1` for the issue `Time-79`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Time-79
//
// ## Issue-Title:
// none standard PeriodType without year throws exception
//
// ## Issue-Description:
// Hi.
//
//
// I tried to get a Period only for months and weeks with following code:
//
//
//
// ```
// Period p = new Period(new DateTime(startDate.getTime()), new DateTime(endDate.getTime()), PeriodType.forFields(new DurationFieldType[]{DurationFieldType.months(), DurationFieldType.weeks()})).normalizedStandard(PeriodType.forFields(new DurationFieldType[]{DurationFieldType.months(), DurationFieldType.weeks()}));
// return p.getMonths();
// ```
//
// This throws following exception:
//
//
//
// ```
// 10-17 14:35:50.999: E/AndroidRuntime(1350): java.lang.UnsupportedOperationException: Field is not supported
// 10-17 14:35:50.999: E/AndroidRuntime(1350): at org.joda.time.PeriodType.setIndexedField(PeriodType.java:690)
// 10-17 14:35:50.999: E/AndroidRuntime(1350): at org.joda.time.Period.withYears(Period.java:896) 10-17
// 14:35:50.999: E/AndroidRuntime(1350): at org.joda.time.Period.normalizedStandard(Period.java:1630)
//
// ```
//
// Even removing the year component with .withYearsRemoved() throws the same exception:
//
//
// this works:
//
//
//
// ```
// Period p = new Period(new DateTime(startDate.getTime()), new DateTime(endDate.getTime()), PeriodType.standard()).normalizedStandard(PeriodType.standard());
// return p.getMonths();
// ```
//
// this fails:
//
//
//
// ```
// Period p = new Period(new DateTime(startDate.getTime()), new DateTime(endDate.getTime()), PeriodType.standard().withYearsRemoved()).normalizedStandard(PeriodType.standard().withYearsRemoved());
// return p.getMonths();
// ```
//
//
//
| Time | /*
* Copyright 2001-2005 Stephen Colebourne
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.joda.time;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.math.BigInteger;
import java.util.Arrays;
import java.util.Locale;
import java.util.TimeZone;
import junit.framework.TestCase;
import junit.framework.TestSuite;
import org.joda.time.base.BasePeriod;
import org.joda.time.format.PeriodFormat;
import org.joda.time.format.PeriodFormatter;
/**
* This class is a Junit unit test for Duration.
*
* @author Stephen Colebourne
*/
public class TestPeriod_Basics extends TestCase {
// Test in 2002/03 as time zones are more well known
// (before the late 90's they were all over the place)
//private static final DateTimeZone PARIS = DateTimeZone.forID("Europe/Paris");
private static final DateTimeZone LONDON = DateTimeZone.forID("Europe/London");
long y2002days = 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 +
365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365;
long y2003days = 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 +
365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365 + 365;
// 2002-06-09
private long TEST_TIME_NOW =
(y2002days + 31L + 28L + 31L + 30L + 31L + 9L -1L) * DateTimeConstants.MILLIS_PER_DAY;
// 2002-04-05
private long TEST_TIME1 =
(y2002days + 31L + 28L + 31L + 5L -1L) * DateTimeConstants.MILLIS_PER_DAY
+ 12L * DateTimeConstants.MILLIS_PER_HOUR
+ 24L * DateTimeConstants.MILLIS_PER_MINUTE;
// 2003-05-06
private long TEST_TIME2 =
(y2003days + 31L + 28L + 31L + 30L + 6L -1L) * DateTimeConstants.MILLIS_PER_DAY
+ 14L * DateTimeConstants.MILLIS_PER_HOUR
+ 28L * DateTimeConstants.MILLIS_PER_MINUTE;
private DateTimeZone originalDateTimeZone = null;
private TimeZone originalTimeZone = null;
private Locale originalLocale = null;
public static void main(String[] args) {
junit.textui.TestRunner.run(suite());
}
public static TestSuite suite() {
return new TestSuite(TestPeriod_Basics.class);
}
public TestPeriod_Basics(String name) {
super(name);
}
protected void setUp() throws Exception {
DateTimeUtils.setCurrentMillisFixed(TEST_TIME_NOW);
originalDateTimeZone = DateTimeZone.getDefault();
originalTimeZone = TimeZone.getDefault();
originalLocale = Locale.getDefault();
DateTimeZone.setDefault(LONDON);
TimeZone.setDefault(TimeZone.getTimeZone("Europe/London"));
Locale.setDefault(Locale.UK);
}
protected void tearDown() throws Exception {
DateTimeUtils.setCurrentMillisSystem();
DateTimeZone.setDefault(originalDateTimeZone);
TimeZone.setDefault(originalTimeZone);
Locale.setDefault(originalLocale);
originalDateTimeZone = null;
originalTimeZone = null;
originalLocale = null;
}
//-----------------------------------------------------------------------
public void testTest() {
assertEquals("2002-06-09T00:00:00.000Z", new Instant(TEST_TIME_NOW).toString());
assertEquals("2002-04-05T12:24:00.000Z", new Instant(TEST_TIME1).toString());
assertEquals("2003-05-06T14:28:00.000Z", new Instant(TEST_TIME2).toString());
}
//-----------------------------------------------------------------------
public void testGetPeriodType() {
Period test = new Period(0L);
assertEquals(PeriodType.standard(), test.getPeriodType());
}
public void testGetMethods() {
Period test = new Period(0L);
assertEquals(0, test.getYears());
assertEquals(0, test.getMonths());
assertEquals(0, test.getWeeks());
assertEquals(0, test.getDays());
assertEquals(0, test.getHours());
assertEquals(0, test.getMinutes());
assertEquals(0, test.getSeconds());
assertEquals(0, test.getMillis());
}
public void testValueIndexMethods() {
Period test = new Period(1, 0, 0, 4, 5, 6, 7, 8, PeriodType.yearDayTime());
assertEquals(6, test.size());
assertEquals(1, test.getValue(0));
assertEquals(4, test.getValue(1));
assertEquals(5, test.getValue(2));
assertEquals(6, test.getValue(3));
assertEquals(7, test.getValue(4));
assertEquals(8, test.getValue(5));
assertEquals(true, Arrays.equals(new int[] {1, 4, 5, 6, 7, 8}, test.getValues()));
}
public void testTypeIndexMethods() {
Period test = new Period(1, 0, 0, 4, 5, 6, 7, 8, PeriodType.yearDayTime());
assertEquals(6, test.size());
assertEquals(DurationFieldType.years(), test.getFieldType(0));
assertEquals(DurationFieldType.days(), test.getFieldType(1));
assertEquals(DurationFieldType.hours(), test.getFieldType(2));
assertEquals(DurationFieldType.minutes(), test.getFieldType(3));
assertEquals(DurationFieldType.seconds(), test.getFieldType(4));
assertEquals(DurationFieldType.millis(), test.getFieldType(5));
assertEquals(true, Arrays.equals(new DurationFieldType[] {
DurationFieldType.years(), DurationFieldType.days(), DurationFieldType.hours(),
DurationFieldType.minutes(), DurationFieldType.seconds(), DurationFieldType.millis()},
test.getFieldTypes()));
}
public void testIsSupported() {
Period test = new Period(1, 0, 0, 4, 5, 6, 7, 8, PeriodType.yearDayTime());
assertEquals(true, test.isSupported(DurationFieldType.years()));
assertEquals(false, test.isSupported(DurationFieldType.months()));
assertEquals(false, test.isSupported(DurationFieldType.weeks()));
assertEquals(true, test.isSupported(DurationFieldType.days()));
assertEquals(true, test.isSupported(DurationFieldType.hours()));
assertEquals(true, test.isSupported(DurationFieldType.minutes()));
assertEquals(true, test.isSupported(DurationFieldType.seconds()));
assertEquals(true, test.isSupported(DurationFieldType.millis()));
}
public void testIndexOf() {
Period test = new Period(1, 0, 0, 4, 5, 6, 7, 8, PeriodType.yearDayTime());
assertEquals(0, test.indexOf(DurationFieldType.years()));
assertEquals(-1, test.indexOf(DurationFieldType.months()));
assertEquals(-1, test.indexOf(DurationFieldType.weeks()));
assertEquals(1, test.indexOf(DurationFieldType.days()));
assertEquals(2, test.indexOf(DurationFieldType.hours()));
assertEquals(3, test.indexOf(DurationFieldType.minutes()));
assertEquals(4, test.indexOf(DurationFieldType.seconds()));
assertEquals(5, test.indexOf(DurationFieldType.millis()));
}
public void testGet() {
Period test = new Period(1, 0, 0, 4, 5, 6, 7, 8, PeriodType.yearDayTime());
assertEquals(1, test.get(DurationFieldType.years()));
assertEquals(0, test.get(DurationFieldType.months()));
assertEquals(0, test.get(DurationFieldType.weeks()));
assertEquals(4, test.get(DurationFieldType.days()));
assertEquals(5, test.get(DurationFieldType.hours()));
assertEquals(6, test.get(DurationFieldType.minutes()));
assertEquals(7, test.get(DurationFieldType.seconds()));
assertEquals(8, test.get(DurationFieldType.millis()));
}
public void testEqualsHashCode() {
Period test1 = new Period(123L);
Period test2 = new Period(123L);
assertEquals(true, test1.equals(test2));
assertEquals(true, test2.equals(test1));
assertEquals(true, test1.equals(test1));
assertEquals(true, test2.equals(test2));
assertEquals(true, test1.hashCode() == test2.hashCode());
assertEquals(true, test1.hashCode() == test1.hashCode());
assertEquals(true, test2.hashCode() == test2.hashCode());
Period test3 = new Period(321L);
assertEquals(false, test1.equals(test3));
assertEquals(false, test2.equals(test3));
assertEquals(false, test3.equals(test1));
assertEquals(false, test3.equals(test2));
assertEquals(false, test1.hashCode() == test3.hashCode());
assertEquals(false, test2.hashCode() == test3.hashCode());
assertEquals(false, test1.equals("Hello"));
assertEquals(true, test1.equals(new MockPeriod(123L)));
assertEquals(false, test1.equals(new Period(123L, PeriodType.dayTime())));
}
class MockPeriod extends BasePeriod {
private static final long serialVersionUID = 1L;
public MockPeriod(long value) {
super(value, null, null);
}
}
//-----------------------------------------------------------------------
public void testSerialization() throws Exception {
Period test = new Period(123L);
ByteArrayOutputStream baos = new ByteArrayOutputStream();
ObjectOutputStream oos = new ObjectOutputStream(baos);
oos.writeObject(test);
byte[] bytes = baos.toByteArray();
oos.close();
ByteArrayInputStream bais = new ByteArrayInputStream(bytes);
ObjectInputStream ois = new ObjectInputStream(bais);
Period result = (Period) ois.readObject();
ois.close();
assertEquals(test, result);
}
// //-----------------------------------------------------------------------
// public void testAddTo1() {
// long expected = TEST_TIME_NOW;
// expected = ISOChronology.getInstance().years().add(expected, 1);
// expected = ISOChronology.getInstance().months().add(expected, 2);
// expected = ISOChronology.getInstance().weeks().add(expected, 3);
// expected = ISOChronology.getInstance().days().add(expected, 4);
// expected = ISOChronology.getInstance().hours().add(expected, 5);
// expected = ISOChronology.getInstance().minutes().add(expected, 6);
// expected = ISOChronology.getInstance().seconds().add(expected, 7);
// expected = ISOChronology.getInstance().millis().add(expected, 8);
//
// Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8);
// long added = test.addTo(TEST_TIME_NOW, 1);
// assertEquals(expected, added);
// }
//
// public void testAddTo2() {
// long expected = TEST_TIME_NOW;
// expected = ISOChronology.getInstance().years().add(expected, -2);
// expected = ISOChronology.getInstance().months().add(expected, -4);
// expected = ISOChronology.getInstance().weeks().add(expected, -6);
// expected = ISOChronology.getInstance().days().add(expected, -8);
// expected = ISOChronology.getInstance().hours().add(expected, -10);
// expected = ISOChronology.getInstance().minutes().add(expected, -12);
// expected = ISOChronology.getInstance().seconds().add(expected, -14);
// expected = ISOChronology.getInstance().millis().add(expected, -16);
//
// Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8);
// long added = test.addTo(TEST_TIME_NOW, -2);
// assertEquals(expected, added);
// }
//
// public void testAddTo3() {
// long expected = TEST_TIME_NOW;
// Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8);
// long added = test.addTo(TEST_TIME_NOW, 0);
// assertEquals(expected, added);
// }
//
// public void testAddTo4() {
// long expected = TEST_TIME_NOW + 100L;
// Period test = new Period(100L);
// long added = test.addTo(TEST_TIME_NOW, 1);
// assertEquals(expected, added);
// }
//
// //-----------------------------------------------------------------------
// public void testAddToWithChronology1() {
// long expected = TEST_TIME_NOW;
// expected = ISOChronology.getInstance().years().add(expected, 1);
// expected = ISOChronology.getInstance().months().add(expected, 2);
// expected = ISOChronology.getInstance().weeks().add(expected, 3);
// expected = ISOChronology.getInstance().days().add(expected, 4);
// expected = ISOChronology.getInstance().hours().add(expected, 5);
// expected = ISOChronology.getInstance().minutes().add(expected, 6);
// expected = ISOChronology.getInstance().seconds().add(expected, 7);
// expected = ISOChronology.getInstance().millis().add(expected, 8);
//
// Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8);
// long added = test.addTo(TEST_TIME_NOW, 1, ISOChronology.getInstance());
// assertEquals(expected, added);
// }
//
// public void testAddToWithChronology2() {
// long expected = TEST_TIME_NOW;
// expected = ISOChronology.getInstanceUTC().years().add(expected, -2);
// expected = ISOChronology.getInstanceUTC().months().add(expected, -4);
// expected = ISOChronology.getInstanceUTC().weeks().add(expected, -6);
// expected = ISOChronology.getInstanceUTC().days().add(expected, -8);
// expected = ISOChronology.getInstanceUTC().hours().add(expected, -10);
// expected = ISOChronology.getInstanceUTC().minutes().add(expected, -12);
// expected = ISOChronology.getInstanceUTC().seconds().add(expected, -14);
// expected = ISOChronology.getInstanceUTC().millis().add(expected, -16);
//
// Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8, PeriodType.standard());
// long added = test.addTo(TEST_TIME_NOW, -2, ISOChronology.getInstanceUTC()); // local specified so use it
// assertEquals(expected, added);
// }
//
// public void testAddToWithChronology3() {
// long expected = TEST_TIME_NOW;
// expected = ISOChronology.getInstance().years().add(expected, -2);
// expected = ISOChronology.getInstance().months().add(expected, -4);
// expected = ISOChronology.getInstance().weeks().add(expected, -6);
// expected = ISOChronology.getInstance().days().add(expected, -8);
// expected = ISOChronology.getInstance().hours().add(expected, -10);
// expected = ISOChronology.getInstance().minutes().add(expected, -12);
// expected = ISOChronology.getInstance().seconds().add(expected, -14);
// expected = ISOChronology.getInstance().millis().add(expected, -16);
//
// Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8, PeriodType.standard());
// long added = test.addTo(TEST_TIME_NOW, -2, null); // no chrono specified so use default
// assertEquals(expected, added);
// }
//
// //-----------------------------------------------------------------------
// public void testAddToRI1() {
// long expected = TEST_TIME_NOW;
// expected = ISOChronology.getInstance().years().add(expected, 1);
// expected = ISOChronology.getInstance().months().add(expected, 2);
// expected = ISOChronology.getInstance().weeks().add(expected, 3);
// expected = ISOChronology.getInstance().days().add(expected, 4);
// expected = ISOChronology.getInstance().hours().add(expected, 5);
// expected = ISOChronology.getInstance().minutes().add(expected, 6);
// expected = ISOChronology.getInstance().seconds().add(expected, 7);
// expected = ISOChronology.getInstance().millis().add(expected, 8);
//
// Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8);
// DateTime added = test.addTo(new Instant(), 1); // Instant has no time zone, use default
// assertEquals(expected, added.getMillis());
// assertEquals(ISOChronology.getInstance(), added.getChronology());
// }
//
// public void testAddToRI2() {
// long expected = TEST_TIME_NOW;
// expected = ISOChronology.getInstance().years().add(expected, -2);
// expected = ISOChronology.getInstance().months().add(expected, -4);
// expected = ISOChronology.getInstance().weeks().add(expected, -6);
// expected = ISOChronology.getInstance().days().add(expected, -8);
// expected = ISOChronology.getInstance().hours().add(expected, -10);
// expected = ISOChronology.getInstance().minutes().add(expected, -12);
// expected = ISOChronology.getInstance().seconds().add(expected, -14);
// expected = ISOChronology.getInstance().millis().add(expected, -16);
//
// Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8, PeriodType.standard());
// DateTime added = test.addTo(new Instant(), -2); // Instant has no time zone, use default
// assertEquals(expected, added.getMillis());
// assertEquals(ISOChronology.getInstance(), added.getChronology());
// }
//
// public void testAddToRI3() {
// long expected = TEST_TIME_NOW;
// expected = ISOChronology.getInstanceUTC().years().add(expected, -2);
// expected = ISOChronology.getInstanceUTC().months().add(expected, -4);
// expected = ISOChronology.getInstanceUTC().weeks().add(expected, -6);
// expected = ISOChronology.getInstanceUTC().days().add(expected, -8);
// expected = ISOChronology.getInstanceUTC().hours().add(expected, -10);
// expected = ISOChronology.getInstanceUTC().minutes().add(expected, -12);
// expected = ISOChronology.getInstanceUTC().seconds().add(expected, -14);
// expected = ISOChronology.getInstanceUTC().millis().add(expected, -16);
//
// Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8, PeriodType.standard());
// DateTime added = test.addTo(new DateTime(ISOChronology.getInstanceUTC()), -2); // DateTime has UTC time zone
// assertEquals(expected, added.getMillis());
// assertEquals(ISOChronology.getInstanceUTC(), added.getChronology());
// }
//
// public void testAddToRI4() {
// long expected = TEST_TIME_NOW;
// expected = ISOChronology.getInstance(PARIS).years().add(expected, -2);
// expected = ISOChronology.getInstance(PARIS).months().add(expected, -4);
// expected = ISOChronology.getInstance(PARIS).weeks().add(expected, -6);
// expected = ISOChronology.getInstance(PARIS).days().add(expected, -8);
// expected = ISOChronology.getInstance(PARIS).hours().add(expected, -10);
// expected = ISOChronology.getInstance(PARIS).minutes().add(expected, -12);
// expected = ISOChronology.getInstance(PARIS).seconds().add(expected, -14);
// expected = ISOChronology.getInstance(PARIS).millis().add(expected, -16);
//
// Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8, PeriodType.standard());
// DateTime added = test.addTo(new DateTime(PARIS), -2); // DateTime has PARIS time zone
// assertEquals(expected, added.getMillis());
// assertEquals(ISOChronology.getInstance(PARIS), added.getChronology());
// }
//
// public void testAddToRI5() {
// long expected = TEST_TIME_NOW;
// expected = ISOChronology.getInstance().years().add(expected, -2);
// expected = ISOChronology.getInstance().months().add(expected, -4);
// expected = ISOChronology.getInstance().weeks().add(expected, -6);
// expected = ISOChronology.getInstance().days().add(expected, -8);
// expected = ISOChronology.getInstance().hours().add(expected, -10);
// expected = ISOChronology.getInstance().minutes().add(expected, -12);
// expected = ISOChronology.getInstance().seconds().add(expected, -14);
// expected = ISOChronology.getInstance().millis().add(expected, -16);
//
// Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8, PeriodType.standard());
// DateTime added = test.addTo(null, -2); // null has no time zone, use default
// assertEquals(expected, added.getMillis());
// assertEquals(ISOChronology.getInstance(), added.getChronology());
// }
//
// //-----------------------------------------------------------------------
// public void testAddIntoRWI1() {
// long expected = TEST_TIME_NOW;
// expected = ISOChronology.getInstance().years().add(expected, 1);
// expected = ISOChronology.getInstance().months().add(expected, 2);
// expected = ISOChronology.getInstance().weeks().add(expected, 3);
// expected = ISOChronology.getInstance().days().add(expected, 4);
// expected = ISOChronology.getInstance().hours().add(expected, 5);
// expected = ISOChronology.getInstance().minutes().add(expected, 6);
// expected = ISOChronology.getInstance().seconds().add(expected, 7);
// expected = ISOChronology.getInstance().millis().add(expected, 8);
//
// Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8);
// MutableDateTime mdt = new MutableDateTime();
// test.addInto(mdt, 1);
// assertEquals(expected, mdt.getMillis());
// }
//
// public void testAddIntoRWI2() {
// long expected = TEST_TIME_NOW;
// expected = ISOChronology.getInstance().years().add(expected, -2);
// expected = ISOChronology.getInstance().months().add(expected, -4);
// expected = ISOChronology.getInstance().weeks().add(expected, -6);
// expected = ISOChronology.getInstance().days().add(expected, -8);
// expected = ISOChronology.getInstance().hours().add(expected, -10);
// expected = ISOChronology.getInstance().minutes().add(expected, -12);
// expected = ISOChronology.getInstance().seconds().add(expected, -14);
// expected = ISOChronology.getInstance().millis().add(expected, -16);
//
// Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8, PeriodType.standard());
// MutableDateTime mdt = new MutableDateTime();
// test.addInto(mdt, -2); // MutableDateTime has a chronology, use it
// assertEquals(expected, mdt.getMillis());
// }
//
// public void testAddIntoRWI3() {
// Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8);
// try {
// test.addInto(null, 1);
// fail();
// } catch (IllegalArgumentException ex) {}
// }
//-----------------------------------------------------------------------
public void testToString() {
Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8);
assertEquals("P1Y2M3W4DT5H6M7.008S", test.toString());
test = new Period(0, 0, 0, 0, 0, 0, 0, 0);
assertEquals("PT0S", test.toString());
test = new Period(12345L);
assertEquals("PT12.345S", test.toString());
}
//-----------------------------------------------------------------------
public void testToString_PeriodFormatter() {
Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8);
assertEquals("1 year, 2 months, 3 weeks, 4 days, 5 hours, 6 minutes, 7 seconds and 8 milliseconds", test.toString(PeriodFormat.getDefault()));
test = new Period(0, 0, 0, 0, 0, 0, 0, 0);
assertEquals("0 milliseconds", test.toString(PeriodFormat.getDefault()));
}
public void testToString_nullPeriodFormatter() {
Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8);
assertEquals("P1Y2M3W4DT5H6M7.008S", test.toString((PeriodFormatter) null));
}
//-----------------------------------------------------------------------
public void testToPeriod() {
Period test = new Period(123L);
Period result = test.toPeriod();
assertSame(test, result);
}
public void testToMutablePeriod() {
Period test = new Period(123L);
MutablePeriod result = test.toMutablePeriod();
assertEquals(test, result);
}
//-----------------------------------------------------------------------
// public void testToDurationMillisFrom() {
// Period test = new Period(123L);
// assertEquals(123L, test.toDurationMillisFrom(0L, null));
// }
public void testToDurationFrom() {
Period test = new Period(123L);
assertEquals(new Duration(123L), test.toDurationFrom(new Instant(0L)));
}
public void testToDurationTo() {
Period test = new Period(123L);
assertEquals(new Duration(123L), test.toDurationTo(new Instant(123L)));
}
//-----------------------------------------------------------------------
public void testWithPeriodType1() {
Period test = new Period(123L);
Period result = test.withPeriodType(PeriodType.standard());
assertSame(test, result);
}
public void testWithPeriodType2() {
Period test = new Period(3123L);
Period result = test.withPeriodType(PeriodType.dayTime());
assertEquals(3, result.getSeconds());
assertEquals(123, result.getMillis());
assertEquals(PeriodType.dayTime(), result.getPeriodType());
}
public void testWithPeriodType3() {
Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8, PeriodType.standard());
try {
test.withPeriodType(PeriodType.dayTime());
fail();
} catch (IllegalArgumentException ex) {}
}
public void testWithPeriodType4() {
Period test = new Period(3123L);
Period result = test.withPeriodType(null);
assertEquals(3, result.getSeconds());
assertEquals(123, result.getMillis());
assertEquals(PeriodType.standard(), result.getPeriodType());
}
public void testWithPeriodType5() {
Period test = new Period(1, 2, 0, 4, 5, 6, 7, 8, PeriodType.standard());
Period result = test.withPeriodType(PeriodType.yearMonthDayTime());
assertEquals(PeriodType.yearMonthDayTime(), result.getPeriodType());
assertEquals(1, result.getYears());
assertEquals(2, result.getMonths());
assertEquals(0, result.getWeeks());
assertEquals(4, result.getDays());
assertEquals(5, result.getHours());
assertEquals(6, result.getMinutes());
assertEquals(7, result.getSeconds());
assertEquals(8, result.getMillis());
}
//-----------------------------------------------------------------------
public void testWithFields1() {
Period test1 = new Period(1, 2, 3, 4, 5, 6, 7, 8);
Period test2 = new Period(0, 0, 0, 0, 0, 0, 0, 9, PeriodType.millis());
Period result = test1.withFields(test2);
assertEquals(new Period(1, 2, 3, 4, 5, 6, 7, 8), test1);
assertEquals(new Period(0, 0, 0, 0, 0, 0, 0, 9, PeriodType.millis()), test2);
assertEquals(new Period(1, 2, 3, 4, 5, 6, 7, 9), result);
}
public void testWithFields2() {
Period test1 = new Period(1, 2, 3, 4, 5, 6, 7, 8);
Period test2 = null;
Period result = test1.withFields(test2);
assertEquals(new Period(1, 2, 3, 4, 5, 6, 7, 8), test1);
assertSame(test1, result);
}
public void testWithFields3() {
Period test1 = new Period(0, 0, 0, 0, 0, 0, 0, 9, PeriodType.millis());
Period test2 = new Period(1, 2, 3, 4, 5, 6, 7, 8);
try {
test1.withFields(test2);
fail();
} catch (IllegalArgumentException ex) {}
assertEquals(new Period(0, 0, 0, 0, 0, 0, 0, 9, PeriodType.millis()), test1);
assertEquals(new Period(1, 2, 3, 4, 5, 6, 7, 8), test2);
}
//-----------------------------------------------------------------------
public void testWithField1() {
Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8);
Period result = test.withField(DurationFieldType.years(), 6);
assertEquals(new Period(1, 2, 3, 4, 5, 6, 7, 8), test);
assertEquals(new Period(6, 2, 3, 4, 5, 6, 7, 8), result);
}
public void testWithField2() {
Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8);
try {
test.withField(null, 6);
fail();
} catch (IllegalArgumentException ex) {}
}
public void testWithField3() {
Period test = new Period(0, 0, 0, 0, 5, 6, 7, 8, PeriodType.time());
try {
test.withField(DurationFieldType.years(), 6);
fail();
} catch (IllegalArgumentException ex) {}
}
public void testWithField4() {
Period test = new Period(0, 0, 0, 0, 5, 6, 7, 8, PeriodType.time());
Period result = test.withField(DurationFieldType.years(), 0);
assertEquals(test, result);
}
//-----------------------------------------------------------------------
public void testWithFieldAdded1() {
Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8);
Period result = test.withFieldAdded(DurationFieldType.years(), 6);
assertEquals(new Period(1, 2, 3, 4, 5, 6, 7, 8), test);
assertEquals(new Period(7, 2, 3, 4, 5, 6, 7, 8), result);
}
public void testWithFieldAdded2() {
Period test = new Period(1, 2, 3, 4, 5, 6, 7, 8);
try {
test.withFieldAdded(null, 0);
fail();
} catch (IllegalArgumentException ex) {}
}
public void testWithFieldAdded3() {
Period test = new Period(0, 0, 0, 0, 5, 6, 7, 8, PeriodType.time());
try {
test.withFieldAdded(DurationFieldType.years(), 6);
fail();
} catch (IllegalArgumentException ex) {}
}
public void testWithFieldAdded4() {
Period test = new Period(0, 0, 0, 0, 5, 6, 7, 8, PeriodType.time());
Period result = test.withFieldAdded(DurationFieldType.years(), 0);
assertEquals(test, result);
}
//-----------------------------------------------------------------------
public void testPeriodStatics() {
Period test;
test = Period.years(1);
assertEquals(test, new Period(1, 0, 0, 0, 0, 0, 0, 0, PeriodType.standard()));
test = Period.months(1);
assertEquals(test, new Period(0, 1, 0, 0, 0, 0, 0, 0, PeriodType.standard()));
test = Period.weeks(1);
assertEquals(test, new Period(0, 0, 1, 0, 0, 0, 0, 0, PeriodType.standard()));
test = Period.days(1);
assertEquals(test, new Period(0, 0, 0, 1, 0, 0, 0, 0, PeriodType.standard()));
test = Period.hours(1);
assertEquals(test, new Period(0, 0, 0, 0, 1, 0, 0, 0, PeriodType.standard()));
test = Period.minutes(1);
assertEquals(test, new Period(0, 0, 0, 0, 0, 1, 0, 0, PeriodType.standard()));
test = Period.seconds(1);
assertEquals(test, new Period(0, 0, 0, 0, 0, 0, 1, 0, PeriodType.standard()));
test = Period.millis(1);
assertEquals(test, new Period(0, 0, 0, 0, 0, 0, 0, 1, PeriodType.standard()));
}
//-----------------------------------------------------------------------
public void testWith() {
Period test;
test = Period.years(5).withYears(1);
assertEquals(test, new Period(1, 0, 0, 0, 0, 0, 0, 0, PeriodType.standard()));
test = Period.months(5).withMonths(1);
assertEquals(test, new Period(0, 1, 0, 0, 0, 0, 0, 0, PeriodType.standard()));
test = Period.weeks(5).withWeeks(1);
assertEquals(test, new Period(0, 0, 1, 0, 0, 0, 0, 0, PeriodType.standard()));
test = Period.days(5).withDays(1);
assertEquals(test, new Period(0, 0, 0, 1, 0, 0, 0, 0, PeriodType.standard()));
test = Period.hours(5).withHours(1);
assertEquals(test, new Period(0, 0, 0, 0, 1, 0, 0, 0, PeriodType.standard()));
test = Period.minutes(5).withMinutes(1);
assertEquals(test, new Period(0, 0, 0, 0, 0, 1, 0, 0, PeriodType.standard()));
test = Period.seconds(5).withSeconds(1);
assertEquals(test, new Period(0, 0, 0, 0, 0, 0, 1, 0, PeriodType.standard()));
test = Period.millis(5).withMillis(1);
assertEquals(test, new Period(0, 0, 0, 0, 0, 0, 0, 1, PeriodType.standard()));
test = new Period(0L, PeriodType.millis());
try {
test.withYears(1);
fail();
} catch (UnsupportedOperationException ex) {}
}
//-----------------------------------------------------------------------
public void testPlus() {
Period base = new Period(1, 2, 3, 4, 5, 6, 7, 8);
Period baseDaysOnly = new Period(0, 0, 0, 10, 0, 0, 0, 0, PeriodType.days());
Period test = base.plus((ReadablePeriod) null);
assertSame(base, test);
test = base.plus(Period.years(10));
assertEquals(11, test.getYears());
assertEquals(2, test.getMonths());
assertEquals(3, test.getWeeks());
assertEquals(4, test.getDays());
assertEquals(5, test.getHours());
assertEquals(6, test.getMinutes());
assertEquals(7, test.getSeconds());
assertEquals(8, test.getMillis());
test = base.plus(Years.years(10));
assertEquals(11, test.getYears());
assertEquals(2, test.getMonths());
assertEquals(3, test.getWeeks());
assertEquals(4, test.getDays());
assertEquals(5, test.getHours());
assertEquals(6, test.getMinutes());
assertEquals(7, test.getSeconds());
assertEquals(8, test.getMillis());
test = base.plus(Period.days(10));
assertEquals(1, test.getYears());
assertEquals(2, test.getMonths());
assertEquals(3, test.getWeeks());
assertEquals(14, test.getDays());
assertEquals(5, test.getHours());
assertEquals(6, test.getMinutes());
assertEquals(7, test.getSeconds());
assertEquals(8, test.getMillis());
test = baseDaysOnly.plus(Period.years(0));
assertEquals(0, test.getYears());
assertEquals(0, test.getMonths());
assertEquals(0, test.getWeeks());
assertEquals(10, test.getDays());
assertEquals(0, test.getHours());
assertEquals(0, test.getMinutes());
assertEquals(0, test.getSeconds());
assertEquals(0, test.getMillis());
test = baseDaysOnly.plus(baseDaysOnly);
assertEquals(0, test.getYears());
assertEquals(0, test.getMonths());
assertEquals(0, test.getWeeks());
assertEquals(20, test.getDays());
assertEquals(0, test.getHours());
assertEquals(0, test.getMinutes());
assertEquals(0, test.getSeconds());
assertEquals(0, test.getMillis());
try {
baseDaysOnly.plus(Period.years(1));
fail();
} catch (UnsupportedOperationException ex) {}
try {
Period.days(Integer.MAX_VALUE).plus(Period.days(1));
fail();
} catch (ArithmeticException ex) {}
try {
Period.days(Integer.MIN_VALUE).plus(Period.days(-1));
fail();
} catch (ArithmeticException ex) {}
}
//-----------------------------------------------------------------------
public void testMinus() {
Period base = new Period(1, 2, 3, 4, 5, 6, 7, 8);
Period baseDaysOnly = new Period(0, 0, 0, 10, 0, 0, 0, 0, PeriodType.days());
Period test = base.minus((ReadablePeriod) null);
assertSame(base, test);
test = base.minus(Period.years(10));
assertEquals(-9, test.getYears());
assertEquals(2, test.getMonths());
assertEquals(3, test.getWeeks());
assertEquals(4, test.getDays());
assertEquals(5, test.getHours());
assertEquals(6, test.getMinutes());
assertEquals(7, test.getSeconds());
assertEquals(8, test.getMillis());
test = base.minus(Years.years(10));
assertEquals(-9, test.getYears());
assertEquals(2, test.getMonths());
assertEquals(3, test.getWeeks());
assertEquals(4, test.getDays());
assertEquals(5, test.getHours());
assertEquals(6, test.getMinutes());
assertEquals(7, test.getSeconds());
assertEquals(8, test.getMillis());
test = base.minus(Period.days(10));
assertEquals(1, test.getYears());
assertEquals(2, test.getMonths());
assertEquals(3, test.getWeeks());
assertEquals(-6, test.getDays());
assertEquals(5, test.getHours());
assertEquals(6, test.getMinutes());
assertEquals(7, test.getSeconds());
assertEquals(8, test.getMillis());
test = baseDaysOnly.minus(Period.years(0));
assertEquals(0, test.getYears());
assertEquals(0, test.getMonths());
assertEquals(0, test.getWeeks());
assertEquals(10, test.getDays());
assertEquals(0, test.getHours());
assertEquals(0, test.getMinutes());
assertEquals(0, test.getSeconds());
assertEquals(0, test.getMillis());
test = baseDaysOnly.minus(baseDaysOnly);
assertEquals(0, test.getYears());
assertEquals(0, test.getMonths());
assertEquals(0, test.getWeeks());
assertEquals(0, test.getDays());
assertEquals(0, test.getHours());
assertEquals(0, test.getMinutes());
assertEquals(0, test.getSeconds());
assertEquals(0, test.getMillis());
try {
baseDaysOnly.minus(Period.years(1));
fail();
} catch (UnsupportedOperationException ex) {}
try {
Period.days(Integer.MAX_VALUE).minus(Period.days(-1));
fail();
} catch (ArithmeticException ex) {}
try {
Period.days(Integer.MIN_VALUE).minus(Period.days(1));
fail();
} catch (ArithmeticException ex) {}
}
//-----------------------------------------------------------------------
public void testPlusFields() {
Period test;
test = Period.years(1).plusYears(1);
assertEquals(new Period(2, 0, 0, 0, 0, 0, 0, 0, PeriodType.standard()), test);
test = Period.months(1).plusMonths(1);
assertEquals(new Period(0, 2, 0, 0, 0, 0, 0, 0, PeriodType.standard()), test);
test = Period.weeks(1).plusWeeks(1);
assertEquals(new Period(0, 0, 2, 0, 0, 0, 0, 0, PeriodType.standard()), test);
test = Period.days(1).plusDays(1);
assertEquals(new Period(0, 0, 0, 2, 0, 0, 0, 0, PeriodType.standard()), test);
test = Period.hours(1).plusHours(1);
assertEquals(new Period(0, 0, 0, 0, 2, 0, 0, 0, PeriodType.standard()), test);
test = Period.minutes(1).plusMinutes(1);
assertEquals(new Period(0, 0, 0, 0, 0, 2, 0, 0, PeriodType.standard()), test);
test = Period.seconds(1).plusSeconds(1);
assertEquals(new Period(0, 0, 0, 0, 0, 0, 2, 0, PeriodType.standard()), test);
test = Period.millis(1).plusMillis(1);
assertEquals(new Period(0, 0, 0, 0, 0, 0, 0, 2, PeriodType.standard()), test);
test = new Period(0L, PeriodType.millis());
try {
test.plusYears(1);
fail();
} catch (UnsupportedOperationException ex) {}
}
public void testPlusFieldsZero() {
Period test, result;
test = Period.years(1);
result = test.plusYears(0);
assertSame(test, result);
test = Period.months(1);
result = test.plusMonths(0);
assertSame(test, result);
test = Period.weeks(1);
result = test.plusWeeks(0);
assertSame(test, result);
test = Period.days(1);
result = test.plusDays(0);
assertSame(test, result);
test = Period.hours(1);
result = test.plusHours(0);
assertSame(test, result);
test = Period.minutes(1);
result = test.plusMinutes(0);
assertSame(test, result);
test = Period.seconds(1);
result = test.plusSeconds(0);
assertSame(test, result);
test = Period.millis(1);
result = test.plusMillis(0);
assertSame(test, result);
}
public void testMinusFields() {
Period test;
test = Period.years(3).minusYears(1);
assertEquals(new Period(2, 0, 0, 0, 0, 0, 0, 0, PeriodType.standard()), test);
test = Period.months(3).minusMonths(1);
assertEquals(new Period(0, 2, 0, 0, 0, 0, 0, 0, PeriodType.standard()), test);
test = Period.weeks(3).minusWeeks(1);
assertEquals(new Period(0, 0, 2, 0, 0, 0, 0, 0, PeriodType.standard()), test);
test = Period.days(3).minusDays(1);
assertEquals(new Period(0, 0, 0, 2, 0, 0, 0, 0, PeriodType.standard()), test);
test = Period.hours(3).minusHours(1);
assertEquals(new Period(0, 0, 0, 0, 2, 0, 0, 0, PeriodType.standard()), test);
test = Period.minutes(3).minusMinutes(1);
assertEquals(new Period(0, 0, 0, 0, 0, 2, 0, 0, PeriodType.standard()), test);
test = Period.seconds(3).minusSeconds(1);
assertEquals(new Period(0, 0, 0, 0, 0, 0, 2, 0, PeriodType.standard()), test);
test = Period.millis(3).minusMillis(1);
assertEquals(new Period(0, 0, 0, 0, 0, 0, 0, 2, PeriodType.standard()), test);
test = new Period(0L, PeriodType.millis());
try {
test.minusYears(1);
fail();
} catch (UnsupportedOperationException ex) {}
}
//-----------------------------------------------------------------------
public void testMultipliedBy() {
Period base = new Period(1, 2, 3, 4, 5, 6, 7, 8);
Period test = base.multipliedBy(1);
assertSame(base, test);
test = base.multipliedBy(0);
assertEquals(Period.ZERO, test);
test = base.multipliedBy(2);
assertEquals(2, test.getYears());
assertEquals(4, test.getMonths());
assertEquals(6, test.getWeeks());
assertEquals(8, test.getDays());
assertEquals(10, test.getHours());
assertEquals(12, test.getMinutes());
assertEquals(14, test.getSeconds());
assertEquals(16, test.getMillis());
test = base.multipliedBy(3);
assertEquals(3, test.getYears());
assertEquals(6, test.getMonths());
assertEquals(9, test.getWeeks());
assertEquals(12, test.getDays());
assertEquals(15, test.getHours());
assertEquals(18, test.getMinutes());
assertEquals(21, test.getSeconds());
assertEquals(24, test.getMillis());
test = base.multipliedBy(-4);
assertEquals(-4, test.getYears());
assertEquals(-8, test.getMonths());
assertEquals(-12, test.getWeeks());
assertEquals(-16, test.getDays());
assertEquals(-20, test.getHours());
assertEquals(-24, test.getMinutes());
assertEquals(-28, test.getSeconds());
assertEquals(-32, test.getMillis());
try {
Period.days(Integer.MAX_VALUE).multipliedBy(2);
fail();
} catch (ArithmeticException ex) {}
try {
Period.days(Integer.MIN_VALUE).multipliedBy(2);
fail();
} catch (ArithmeticException ex) {}
}
//-----------------------------------------------------------------------
public void testNegated() {
Period base = new Period(1, 2, 3, 4, 5, 6, 7, 8);
Period test = Period.ZERO.negated();
assertEquals(Period.ZERO, test);
test = base.negated();
assertEquals(-1, test.getYears());
assertEquals(-2, test.getMonths());
assertEquals(-3, test.getWeeks());
assertEquals(-4, test.getDays());
assertEquals(-5, test.getHours());
assertEquals(-6, test.getMinutes());
assertEquals(-7, test.getSeconds());
assertEquals(-8, test.getMillis());
test = Period.days(Integer.MAX_VALUE).negated();
assertEquals(-Integer.MAX_VALUE, test.getDays());
try {
Period.days(Integer.MIN_VALUE).negated();
fail();
} catch (ArithmeticException ex) {}
}
//-----------------------------------------------------------------------
public void testToStandardWeeks() {
Period test = new Period(0, 0, 3, 4, 5, 6, 7, 8);
assertEquals(3, test.toStandardWeeks().getWeeks());
test = new Period(0, 0, 3, 7, 0, 0, 0, 0);
assertEquals(4, test.toStandardWeeks().getWeeks());
test = new Period(0, 0, 0, 6, 23, 59, 59, 1000);
assertEquals(1, test.toStandardWeeks().getWeeks());
test = new Period(0, 0, Integer.MAX_VALUE, 0, 0, 0, 0, 0);
assertEquals(Integer.MAX_VALUE, test.toStandardWeeks().getWeeks());
test = new Period(0, 0, 0, Integer.MAX_VALUE, Integer.MAX_VALUE, Integer.MAX_VALUE, Integer.MAX_VALUE, Integer.MAX_VALUE);
long intMax = Integer.MAX_VALUE;
BigInteger expected = BigInteger.valueOf(intMax);
expected = expected.add(BigInteger.valueOf(intMax * DateTimeConstants.MILLIS_PER_SECOND));
expected = expected.add(BigInteger.valueOf(intMax * DateTimeConstants.MILLIS_PER_MINUTE));
expected = expected.add(BigInteger.valueOf(intMax * DateTimeConstants.MILLIS_PER_HOUR));
expected = expected.add(BigInteger.valueOf(intMax * DateTimeConstants.MILLIS_PER_DAY));
expected = expected.divide(BigInteger.valueOf(DateTimeConstants.MILLIS_PER_WEEK));
assertTrue(expected.compareTo(BigInteger.valueOf(Long.MAX_VALUE)) < 0);
assertEquals(expected.longValue(), test.toStandardWeeks().getWeeks());
test = new Period(0, 0, Integer.MAX_VALUE, 7, 0, 0, 0, 0);
try {
test.toStandardWeeks();
fail();
} catch (ArithmeticException ex) {}
}
public void testToStandardWeeks_years() {
Period test = Period.years(1);
try {
test.toStandardWeeks();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.years(-1);
try {
test.toStandardWeeks();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.years(0);
assertEquals(0, test.toStandardWeeks().getWeeks());
}
public void testToStandardWeeks_months() {
Period test = Period.months(1);
try {
test.toStandardWeeks();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.months(-1);
try {
test.toStandardWeeks();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.months(0);
assertEquals(0, test.toStandardWeeks().getWeeks());
}
//-----------------------------------------------------------------------
public void testToStandardDays() {
Period test = new Period(0, 0, 0, 4, 5, 6, 7, 8);
assertEquals(4, test.toStandardDays().getDays());
test = new Period(0, 0, 1, 4, 0, 0, 0, 0);
assertEquals(11, test.toStandardDays().getDays());
test = new Period(0, 0, 0, 0, 23, 59, 59, 1000);
assertEquals(1, test.toStandardDays().getDays());
test = new Period(0, 0, 0, Integer.MAX_VALUE, 0, 0, 0, 0);
assertEquals(Integer.MAX_VALUE, test.toStandardDays().getDays());
test = new Period(0, 0, 0, 0, Integer.MAX_VALUE, Integer.MAX_VALUE, Integer.MAX_VALUE, Integer.MAX_VALUE);
long intMax = Integer.MAX_VALUE;
BigInteger expected = BigInteger.valueOf(intMax);
expected = expected.add(BigInteger.valueOf(intMax * DateTimeConstants.MILLIS_PER_SECOND));
expected = expected.add(BigInteger.valueOf(intMax * DateTimeConstants.MILLIS_PER_MINUTE));
expected = expected.add(BigInteger.valueOf(intMax * DateTimeConstants.MILLIS_PER_HOUR));
expected = expected.divide(BigInteger.valueOf(DateTimeConstants.MILLIS_PER_DAY));
assertTrue(expected.compareTo(BigInteger.valueOf(Long.MAX_VALUE)) < 0);
assertEquals(expected.longValue(), test.toStandardDays().getDays());
test = new Period(0, 0, 0, Integer.MAX_VALUE, 24, 0, 0, 0);
try {
test.toStandardDays();
fail();
} catch (ArithmeticException ex) {}
}
public void testToStandardDays_years() {
Period test = Period.years(1);
try {
test.toStandardDays();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.years(-1);
try {
test.toStandardDays();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.years(0);
assertEquals(0, test.toStandardDays().getDays());
}
public void testToStandardDays_months() {
Period test = Period.months(1);
try {
test.toStandardDays();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.months(-1);
try {
test.toStandardDays();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.months(0);
assertEquals(0, test.toStandardDays().getDays());
}
//-----------------------------------------------------------------------
public void testToStandardHours() {
Period test = new Period(0, 0, 0, 0, 5, 6, 7, 8);
assertEquals(5, test.toStandardHours().getHours());
test = new Period(0, 0, 0, 1, 5, 0, 0, 0);
assertEquals(29, test.toStandardHours().getHours());
test = new Period(0, 0, 0, 0, 0, 59, 59, 1000);
assertEquals(1, test.toStandardHours().getHours());
test = new Period(0, 0, 0, 0, Integer.MAX_VALUE, 0, 0, 0);
assertEquals(Integer.MAX_VALUE, test.toStandardHours().getHours());
test = new Period(0, 0, 0, 0, 0, Integer.MAX_VALUE, Integer.MAX_VALUE, Integer.MAX_VALUE);
long intMax = Integer.MAX_VALUE;
BigInteger expected = BigInteger.valueOf(intMax);
expected = expected.add(BigInteger.valueOf(intMax * DateTimeConstants.MILLIS_PER_SECOND));
expected = expected.add(BigInteger.valueOf(intMax * DateTimeConstants.MILLIS_PER_MINUTE));
expected = expected.divide(BigInteger.valueOf(DateTimeConstants.MILLIS_PER_HOUR));
assertTrue(expected.compareTo(BigInteger.valueOf(Long.MAX_VALUE)) < 0);
assertEquals(expected.longValue(), test.toStandardHours().getHours());
test = new Period(0, 0, 0, 0, Integer.MAX_VALUE, 60, 0, 0);
try {
test.toStandardHours();
fail();
} catch (ArithmeticException ex) {}
}
public void testToStandardHours_years() {
Period test = Period.years(1);
try {
test.toStandardHours();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.years(-1);
try {
test.toStandardHours();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.years(0);
assertEquals(0, test.toStandardHours().getHours());
}
public void testToStandardHours_months() {
Period test = Period.months(1);
try {
test.toStandardHours();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.months(-1);
try {
test.toStandardHours();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.months(0);
assertEquals(0, test.toStandardHours().getHours());
}
//-----------------------------------------------------------------------
public void testToStandardMinutes() {
Period test = new Period(0, 0, 0, 0, 0, 6, 7, 8);
assertEquals(6, test.toStandardMinutes().getMinutes());
test = new Period(0, 0, 0, 0, 1, 6, 0, 0);
assertEquals(66, test.toStandardMinutes().getMinutes());
test = new Period(0, 0, 0, 0, 0, 0, 59, 1000);
assertEquals(1, test.toStandardMinutes().getMinutes());
test = new Period(0, 0, 0, 0, 0, Integer.MAX_VALUE, 0, 0);
assertEquals(Integer.MAX_VALUE, test.toStandardMinutes().getMinutes());
test = new Period(0, 0, 0, 0, 0, 0, Integer.MAX_VALUE, Integer.MAX_VALUE);
long intMax = Integer.MAX_VALUE;
BigInteger expected = BigInteger.valueOf(intMax);
expected = expected.add(BigInteger.valueOf(intMax * DateTimeConstants.MILLIS_PER_SECOND));
expected = expected.divide(BigInteger.valueOf(DateTimeConstants.MILLIS_PER_MINUTE));
assertTrue(expected.compareTo(BigInteger.valueOf(Long.MAX_VALUE)) < 0);
assertEquals(expected.longValue(), test.toStandardMinutes().getMinutes());
test = new Period(0, 0, 0, 0, 0, Integer.MAX_VALUE, 60, 0);
try {
test.toStandardMinutes();
fail();
} catch (ArithmeticException ex) {}
}
public void testToStandardMinutes_years() {
Period test = Period.years(1);
try {
test.toStandardMinutes();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.years(-1);
try {
test.toStandardMinutes();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.years(0);
assertEquals(0, test.toStandardMinutes().getMinutes());
}
public void testToStandardMinutes_months() {
Period test = Period.months(1);
try {
test.toStandardMinutes();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.months(-1);
try {
test.toStandardMinutes();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.months(0);
assertEquals(0, test.toStandardMinutes().getMinutes());
}
//-----------------------------------------------------------------------
public void testToStandardSeconds() {
Period test = new Period(0, 0, 0, 0, 0, 0, 7, 8);
assertEquals(7, test.toStandardSeconds().getSeconds());
test = new Period(0, 0, 0, 0, 0, 1, 3, 0);
assertEquals(63, test.toStandardSeconds().getSeconds());
test = new Period(0, 0, 0, 0, 0, 0, 0, 1000);
assertEquals(1, test.toStandardSeconds().getSeconds());
test = new Period(0, 0, 0, 0, 0, 0, Integer.MAX_VALUE, 0);
assertEquals(Integer.MAX_VALUE, test.toStandardSeconds().getSeconds());
test = new Period(0, 0, 0, 0, 0, 0, 20, Integer.MAX_VALUE);
long expected = 20;
expected += ((long) Integer.MAX_VALUE) / DateTimeConstants.MILLIS_PER_SECOND;
assertEquals(expected, test.toStandardSeconds().getSeconds());
test = new Period(0, 0, 0, 0, 0, 0, Integer.MAX_VALUE, 1000);
try {
test.toStandardSeconds();
fail();
} catch (ArithmeticException ex) {}
}
public void testToStandardSeconds_years() {
Period test = Period.years(1);
try {
test.toStandardSeconds();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.years(-1);
try {
test.toStandardSeconds();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.years(0);
assertEquals(0, test.toStandardSeconds().getSeconds());
}
public void testToStandardSeconds_months() {
Period test = Period.months(1);
try {
test.toStandardSeconds();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.months(-1);
try {
test.toStandardSeconds();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.months(0);
assertEquals(0, test.toStandardSeconds().getSeconds());
}
//-----------------------------------------------------------------------
public void testToStandardDuration() {
Period test = new Period(0, 0, 0, 0, 0, 0, 0, 8);
assertEquals(8, test.toStandardDuration().getMillis());
test = new Period(0, 0, 0, 0, 0, 0, 1, 20);
assertEquals(1020, test.toStandardDuration().getMillis());
test = new Period(0, 0, 0, 0, 0, 0, 0, Integer.MAX_VALUE);
assertEquals(Integer.MAX_VALUE, test.toStandardDuration().getMillis());
test = new Period(0, 0, 0, 0, 0, 10, 20, Integer.MAX_VALUE);
long expected = Integer.MAX_VALUE;
expected += 10L * ((long) DateTimeConstants.MILLIS_PER_MINUTE);
expected += 20L * ((long) DateTimeConstants.MILLIS_PER_SECOND);
assertEquals(expected, test.toStandardDuration().getMillis());
// proof that overflow does not occur
BigInteger intMax = BigInteger.valueOf(Integer.MAX_VALUE);
BigInteger exp = intMax;
exp = exp.add(intMax.multiply(BigInteger.valueOf(DateTimeConstants.MILLIS_PER_SECOND)));
exp = exp.add(intMax.multiply(BigInteger.valueOf(DateTimeConstants.MILLIS_PER_MINUTE)));
exp = exp.add(intMax.multiply(BigInteger.valueOf(DateTimeConstants.MILLIS_PER_HOUR)));
exp = exp.add(intMax.multiply(BigInteger.valueOf(DateTimeConstants.MILLIS_PER_DAY)));
exp = exp.add(intMax.multiply(BigInteger.valueOf(DateTimeConstants.MILLIS_PER_WEEK)));
assertTrue(exp.compareTo(BigInteger.valueOf(Long.MAX_VALUE)) < 0);
// test = new Period(0, 0, Integer.MAX_VALUE, Integer.MAX_VALUE, Integer.MAX_VALUE, Integer.MAX_VALUE, Integer.MAX_VALUE, Integer.MAX_VALUE);
// try {
// test.toStandardDuration();
// fail();
// } catch (ArithmeticException ex) {}
}
public void testToStandardDuration_years() {
Period test = Period.years(1);
try {
test.toStandardDuration();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.years(-1);
try {
test.toStandardDuration();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.years(0);
assertEquals(0, test.toStandardDuration().getMillis());
}
public void testToStandardDuration_months() {
Period test = Period.months(1);
try {
test.toStandardDuration();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.months(-1);
try {
test.toStandardDuration();
fail();
} catch (UnsupportedOperationException ex) {}
test = Period.months(0);
assertEquals(0, test.toStandardDuration().getMillis());
}
//-----------------------------------------------------------------------
public void testNormalizedStandard_yearMonth1() {
Period test = new Period(1, 15, 0, 0, 0, 0, 0, 0);
Period result = test.normalizedStandard();
assertEquals(new Period(1, 15, 0, 0, 0, 0, 0, 0), test);
assertEquals(new Period(2, 3, 0, 0, 0, 0, 0, 0), result);
}
public void testNormalizedStandard_yearMonth2() {
Period test = new Period(Integer.MAX_VALUE, 15, 0, 0, 0, 0, 0, 0);
try {
test.normalizedStandard();
fail();
} catch (ArithmeticException ex) {}
}
public void testNormalizedStandard_weekDay1() {
Period test = new Period(0, 0, 1, 12, 0, 0, 0, 0);
Period result = test.normalizedStandard();
assertEquals(new Period(0, 0, 1, 12, 0, 0, 0, 0), test);
assertEquals(new Period(0, 0, 2, 5, 0, 0, 0, 0), result);
}
public void testNormalizedStandard_weekDay2() {
Period test = new Period(0, 0, Integer.MAX_VALUE, 7, 0, 0, 0, 0);
try {
test.normalizedStandard();
fail();
} catch (ArithmeticException ex) {}
}
public void testNormalizedStandard_yearMonthWeekDay() {
Period test = new Period(1, 15, 1, 12, 0, 0, 0, 0);
Period result = test.normalizedStandard();
assertEquals(new Period(1, 15, 1, 12, 0, 0, 0, 0), test);
assertEquals(new Period(2, 3, 2, 5, 0, 0, 0, 0), result);
}
public void testNormalizedStandard_yearMonthDay() {
Period test = new Period(1, 15, 0, 36, 0, 0, 0, 0);
Period result = test.normalizedStandard();
assertEquals(new Period(1, 15, 0, 36, 0, 0, 0, 0), test);
assertEquals(new Period(2, 3, 5, 1, 0, 0, 0, 0), result);
}
public void testNormalizedStandard_negative() {
Period test = new Period(0, 0, 0, 0, 2, -10, 0, 0);
Period result = test.normalizedStandard();
assertEquals(new Period(0, 0, 0, 0, 2, -10, 0, 0), test);
assertEquals(new Period(0, 0, 0, 0, 1, 50, 0, 0), result);
}
public void testNormalizedStandard_fullNegative() {
Period test = new Period(0, 0, 0, 0, 1, -70, 0, 0);
Period result = test.normalizedStandard();
assertEquals(new Period(0, 0, 0, 0, 1, -70, 0, 0), test);
assertEquals(new Period(0, 0, 0, 0, 0, -10, 0, 0), result);
}
//-----------------------------------------------------------------------
public void testNormalizedStandard_periodType_yearMonth1() {
Period test = new Period(1, 15, 0, 0, 0, 0, 0, 0);
Period result = test.normalizedStandard((PeriodType) null);
assertEquals(new Period(1, 15, 0, 0, 0, 0, 0, 0), test);
assertEquals(new Period(2, 3, 0, 0, 0, 0, 0, 0), result);
}
public void testNormalizedStandard_periodType_yearMonth2() {
Period test = new Period(Integer.MAX_VALUE, 15, 0, 0, 0, 0, 0, 0);
try {
test.normalizedStandard((PeriodType) null);
fail();
} catch (ArithmeticException ex) {}
}
public void testNormalizedStandard_periodType_yearMonth3() {
Period test = new Period(1, 15, 3, 4, 0, 0, 0, 0);
try {
test.normalizedStandard(PeriodType.dayTime());
fail();
} catch (UnsupportedOperationException ex) {}
}
public void testNormalizedStandard_periodType_weekDay1() {
Period test = new Period(0, 0, 1, 12, 0, 0, 0, 0);
Period result = test.normalizedStandard((PeriodType) null);
assertEquals(new Period(0, 0, 1, 12, 0, 0, 0, 0), test);
assertEquals(new Period(0, 0, 2, 5, 0, 0, 0, 0), result);
}
public void testNormalizedStandard_periodType_weekDay2() {
Period test = new Period(0, 0, Integer.MAX_VALUE, 7, 0, 0, 0, 0);
try {
test.normalizedStandard((PeriodType) null);
fail();
} catch (ArithmeticException ex) {}
}
public void testNormalizedStandard_periodType_weekDay3() {
Period test = new Period(0, 0, 1, 12, 0, 0, 0, 0);
Period result = test.normalizedStandard(PeriodType.dayTime());
assertEquals(new Period(0, 0, 1, 12, 0, 0, 0, 0), test);
assertEquals(new Period(0, 0, 0, 19, 0, 0, 0, 0, PeriodType.dayTime()), result);
}
public void testNormalizedStandard_periodType_yearMonthWeekDay() {
Period test = new Period(1, 15, 1, 12, 0, 0, 0, 0);
Period result = test.normalizedStandard(PeriodType.yearMonthDayTime());
assertEquals(new Period(1, 15, 1, 12, 0, 0, 0, 0), test);
assertEquals(new Period(2, 3, 0, 19, 0, 0, 0, 0, PeriodType.yearMonthDayTime()), result);
}
public void testNormalizedStandard_periodType_yearMonthDay() {
Period test = new Period(1, 15, 0, 36, 27, 0, 0, 0);
Period result = test.normalizedStandard(PeriodType.yearMonthDayTime());
assertEquals(new Period(1, 15, 0, 36, 27, 0, 0, 0), test);
assertEquals(new Period(2, 3, 0, 37, 3, 0, 0, 0, PeriodType.yearMonthDayTime()), result);
}
public void testNormalizedStandard_periodType_months1() {
Period test = new Period(1, 15, 0, 0, 0, 0, 0, 0);
Period result = test.normalizedStandard(PeriodType.months());
assertEquals(new Period(1, 15, 0, 0, 0, 0, 0, 0), test);
assertEquals(new Period(0, 27, 0, 0, 0, 0, 0, 0, PeriodType.months()), result);
}
public void testNormalizedStandard_periodType_months2() {
Period test = new Period(-2, 15, 0, 0, 0, 0, 0, 0);
Period result = test.normalizedStandard(PeriodType.months());
assertEquals(new Period(-2, 15, 0, 0, 0, 0, 0, 0), test);
assertEquals(new Period(0, -9, 0, 0, 0, 0, 0, 0, PeriodType.months()), result);
}
public void testNormalizedStandard_periodType_months3() {
Period test = new Period(0, 4, 0, 0, 0, 0, 0, 0);
Period result = test.normalizedStandard(PeriodType.months());
assertEquals(new Period(0, 4, 0, 0, 0, 0, 0, 0), test);
assertEquals(new Period(0, 4, 0, 0, 0, 0, 0, 0, PeriodType.months()), result);
}
public void testNormalizedStandard_periodType_years() {
Period test = new Period(1, 15, 0, 0, 0, 0, 0, 0);
try {
test.normalizedStandard(PeriodType.years());
fail();
} catch (UnsupportedOperationException ex) {
// expected
}
}
public void testNormalizedStandard_periodType_monthsWeeks() {
PeriodType type = PeriodType.forFields(new DurationFieldType[]{
DurationFieldType.months(),
DurationFieldType.weeks(),
DurationFieldType.days()});
Period test = new Period(2, 4, 6, 0, 0, 0, 0, 0);
Period result = test.normalizedStandard(type);
assertEquals(new Period(2, 4, 6, 0, 0, 0, 0, 0), test);
assertEquals(new Period(0, 28, 6, 0, 0, 0, 0, 0, type), result);
}
} |
||
public void testIssue1107() {
String json = "{\n" +
" \"inBig\": {\n" +
" \"key\": [\n" +
" { \"inSmall\": \"hello\" }\n" +
" ]\n" +
" }\n" +
"}";
BigClass bigClass = new Gson().fromJson(json, BigClass.class);
SmallClass small = bigClass.inBig.get("key").get(0);
assertNotNull(small);
assertEquals("hello", small.inSmall);
} | com.google.gson.functional.CollectionTest::testIssue1107 | gson/src/test/java/com/google/gson/functional/CollectionTest.java | 412 | gson/src/test/java/com/google/gson/functional/CollectionTest.java | testIssue1107 | /*
* Copyright (C) 2008 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.gson.functional;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.HashSet;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.PriorityQueue;
import java.util.Queue;
import java.util.Set;
import java.util.Stack;
import java.util.Vector;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonElement;
import com.google.gson.JsonPrimitive;
import com.google.gson.JsonSerializationContext;
import com.google.gson.JsonSerializer;
import com.google.gson.common.MoreAsserts;
import com.google.gson.common.TestTypes.BagOfPrimitives;
import com.google.gson.reflect.TypeToken;
import junit.framework.TestCase;
/**
* Functional tests for Json serialization and deserialization of collections.
*
* @author Inderjeet Singh
* @author Joel Leitch
*/
public class CollectionTest extends TestCase {
private Gson gson;
@Override
protected void setUp() throws Exception {
super.setUp();
gson = new Gson();
}
public void testTopLevelCollectionOfIntegersSerialization() {
Collection<Integer> target = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9);
Type targetType = new TypeToken<Collection<Integer>>() {}.getType();
String json = gson.toJson(target, targetType);
assertEquals("[1,2,3,4,5,6,7,8,9]", json);
}
public void testTopLevelCollectionOfIntegersDeserialization() {
String json = "[0,1,2,3,4,5,6,7,8,9]";
Type collectionType = new TypeToken<Collection<Integer>>() { }.getType();
Collection<Integer> target = gson.fromJson(json, collectionType);
int[] expected = {0, 1, 2, 3, 4, 5, 6, 7, 8, 9};
MoreAsserts.assertEquals(expected, toIntArray(target));
}
public void testTopLevelListOfIntegerCollectionsDeserialization() throws Exception {
String json = "[[1,2,3],[4,5,6],[7,8,9]]";
Type collectionType = new TypeToken<Collection<Collection<Integer>>>() {}.getType();
List<Collection<Integer>> target = gson.fromJson(json, collectionType);
int[][] expected = new int[3][3];
for (int i = 0; i < 3; ++i) {
int start = (3 * i) + 1;
for (int j = 0; j < 3; ++j) {
expected[i][j] = start + j;
}
}
for (int i = 0; i < 3; i++) {
MoreAsserts.assertEquals(expected[i], toIntArray(target.get(i)));
}
}
public void testLinkedListSerialization() {
List<String> list = new LinkedList<String>();
list.add("a1");
list.add("a2");
Type linkedListType = new TypeToken<LinkedList<String>>() {}.getType();
String json = gson.toJson(list, linkedListType);
assertTrue(json.contains("a1"));
assertTrue(json.contains("a2"));
}
public void testLinkedListDeserialization() {
String json = "['a1','a2']";
Type linkedListType = new TypeToken<LinkedList<String>>() {}.getType();
List<String> list = gson.fromJson(json, linkedListType);
assertEquals("a1", list.get(0));
assertEquals("a2", list.get(1));
}
public void testQueueSerialization() {
Queue<String> queue = new LinkedList<String>();
queue.add("a1");
queue.add("a2");
Type queueType = new TypeToken<Queue<String>>() {}.getType();
String json = gson.toJson(queue, queueType);
assertTrue(json.contains("a1"));
assertTrue(json.contains("a2"));
}
public void testQueueDeserialization() {
String json = "['a1','a2']";
Type queueType = new TypeToken<Queue<String>>() {}.getType();
Queue<String> queue = gson.fromJson(json, queueType);
assertEquals("a1", queue.element());
queue.remove();
assertEquals("a2", queue.element());
}
public void testPriorityQueue() throws Exception {
Type type = new TypeToken<PriorityQueue<Integer>>(){}.getType();
PriorityQueue<Integer> queue = gson.fromJson("[10, 20, 22]", type);
assertEquals(3, queue.size());
String json = gson.toJson(queue);
assertEquals(10, queue.remove().intValue());
assertEquals(20, queue.remove().intValue());
assertEquals(22, queue.remove().intValue());
assertEquals("[10,20,22]", json);
}
public void testVector() {
Type type = new TypeToken<Vector<Integer>>(){}.getType();
Vector<Integer> target = gson.fromJson("[10, 20, 31]", type);
assertEquals(3, target.size());
assertEquals(10, target.get(0).intValue());
assertEquals(20, target.get(1).intValue());
assertEquals(31, target.get(2).intValue());
String json = gson.toJson(target);
assertEquals("[10,20,31]", json);
}
public void testStack() {
Type type = new TypeToken<Stack<Integer>>(){}.getType();
Stack<Integer> target = gson.fromJson("[11, 13, 17]", type);
assertEquals(3, target.size());
String json = gson.toJson(target);
assertEquals(17, target.pop().intValue());
assertEquals(13, target.pop().intValue());
assertEquals(11, target.pop().intValue());
assertEquals("[11,13,17]", json);
}
public void testNullsInListSerialization() {
List<String> list = new ArrayList<String>();
list.add("foo");
list.add(null);
list.add("bar");
String expected = "[\"foo\",null,\"bar\"]";
Type typeOfList = new TypeToken<List<String>>() {}.getType();
String json = gson.toJson(list, typeOfList);
assertEquals(expected, json);
}
public void testNullsInListDeserialization() {
List<String> expected = new ArrayList<String>();
expected.add("foo");
expected.add(null);
expected.add("bar");
String json = "[\"foo\",null,\"bar\"]";
Type expectedType = new TypeToken<List<String>>() {}.getType();
List<String> target = gson.fromJson(json, expectedType);
for (int i = 0; i < expected.size(); ++i) {
assertEquals(expected.get(i), target.get(i));
}
}
public void testCollectionOfObjectSerialization() {
List<Object> target = new ArrayList<Object>();
target.add("Hello");
target.add("World");
assertEquals("[\"Hello\",\"World\"]", gson.toJson(target));
Type type = new TypeToken<List<Object>>() {}.getType();
assertEquals("[\"Hello\",\"World\"]", gson.toJson(target, type));
}
public void testCollectionOfObjectWithNullSerialization() {
List<Object> target = new ArrayList<Object>();
target.add("Hello");
target.add(null);
target.add("World");
assertEquals("[\"Hello\",null,\"World\"]", gson.toJson(target));
Type type = new TypeToken<List<Object>>() {}.getType();
assertEquals("[\"Hello\",null,\"World\"]", gson.toJson(target, type));
}
public void testCollectionOfStringsSerialization() {
List<String> target = new ArrayList<String>();
target.add("Hello");
target.add("World");
assertEquals("[\"Hello\",\"World\"]", gson.toJson(target));
}
public void testCollectionOfBagOfPrimitivesSerialization() {
List<BagOfPrimitives> target = new ArrayList<BagOfPrimitives>();
BagOfPrimitives objA = new BagOfPrimitives(3L, 1, true, "blah");
BagOfPrimitives objB = new BagOfPrimitives(2L, 6, false, "blahB");
target.add(objA);
target.add(objB);
String result = gson.toJson(target);
assertTrue(result.startsWith("["));
assertTrue(result.endsWith("]"));
for (BagOfPrimitives obj : target) {
assertTrue(result.contains(obj.getExpectedJson()));
}
}
public void testCollectionOfStringsDeserialization() {
String json = "[\"Hello\",\"World\"]";
Type collectionType = new TypeToken<Collection<String>>() { }.getType();
Collection<String> target = gson.fromJson(json, collectionType);
assertTrue(target.contains("Hello"));
assertTrue(target.contains("World"));
}
public void testRawCollectionOfIntegersSerialization() {
Collection<Integer> target = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9);
assertEquals("[1,2,3,4,5,6,7,8,9]", gson.toJson(target));
}
@SuppressWarnings("rawtypes")
public void testRawCollectionSerialization() {
BagOfPrimitives bag1 = new BagOfPrimitives();
Collection target = Arrays.asList(bag1, bag1);
String json = gson.toJson(target);
assertTrue(json.contains(bag1.getExpectedJson()));
}
@SuppressWarnings("rawtypes")
public void testRawCollectionDeserializationNotAlllowed() {
String json = "[0,1,2,3,4,5,6,7,8,9]";
Collection integers = gson.fromJson(json, Collection.class);
// JsonReader converts numbers to double by default so we need a floating point comparison
assertEquals(Arrays.asList(0.0, 1.0, 2.0, 3.0, 4.0, 5.0, 6.0, 7.0, 8.0, 9.0), integers);
json = "[\"Hello\", \"World\"]";
Collection strings = gson.fromJson(json, Collection.class);
assertTrue(strings.contains("Hello"));
assertTrue(strings.contains("World"));
}
@SuppressWarnings({"rawtypes", "unchecked"})
public void testRawCollectionOfBagOfPrimitivesNotAllowed() {
BagOfPrimitives bag = new BagOfPrimitives(10, 20, false, "stringValue");
String json = '[' + bag.getExpectedJson() + ',' + bag.getExpectedJson() + ']';
Collection target = gson.fromJson(json, Collection.class);
assertEquals(2, target.size());
for (Object bag1 : target) {
// Gson 2.0 converts raw objects into maps
Map<String, Object> values = (Map<String, Object>) bag1;
assertTrue(values.containsValue(10.0));
assertTrue(values.containsValue(20.0));
assertTrue(values.containsValue("stringValue"));
}
}
public void testWildcardPrimitiveCollectionSerilaization() throws Exception {
Collection<? extends Integer> target = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9);
Type collectionType = new TypeToken<Collection<? extends Integer>>() { }.getType();
String json = gson.toJson(target, collectionType);
assertEquals("[1,2,3,4,5,6,7,8,9]", json);
json = gson.toJson(target);
assertEquals("[1,2,3,4,5,6,7,8,9]", json);
}
public void testWildcardPrimitiveCollectionDeserilaization() throws Exception {
String json = "[1,2,3,4,5,6,7,8,9]";
Type collectionType = new TypeToken<Collection<? extends Integer>>() { }.getType();
Collection<? extends Integer> target = gson.fromJson(json, collectionType);
assertEquals(9, target.size());
assertTrue(target.contains(1));
assertTrue(target.contains(9));
}
public void testWildcardCollectionField() throws Exception {
Collection<BagOfPrimitives> collection = new ArrayList<BagOfPrimitives>();
BagOfPrimitives objA = new BagOfPrimitives(3L, 1, true, "blah");
BagOfPrimitives objB = new BagOfPrimitives(2L, 6, false, "blahB");
collection.add(objA);
collection.add(objB);
ObjectWithWildcardCollection target = new ObjectWithWildcardCollection(collection);
String json = gson.toJson(target);
assertTrue(json.contains(objA.getExpectedJson()));
assertTrue(json.contains(objB.getExpectedJson()));
target = gson.fromJson(json, ObjectWithWildcardCollection.class);
Collection<? extends BagOfPrimitives> deserializedCollection = target.getCollection();
assertEquals(2, deserializedCollection.size());
assertTrue(deserializedCollection.contains(objA));
assertTrue(deserializedCollection.contains(objB));
}
public void testFieldIsArrayList() {
HasArrayListField object = new HasArrayListField();
object.longs.add(1L);
object.longs.add(3L);
String json = gson.toJson(object, HasArrayListField.class);
assertEquals("{\"longs\":[1,3]}", json);
HasArrayListField copy = gson.fromJson("{\"longs\":[1,3]}", HasArrayListField.class);
assertEquals(Arrays.asList(1L, 3L), copy.longs);
}
public void testUserCollectionTypeAdapter() {
Type listOfString = new TypeToken<List<String>>() {}.getType();
Object stringListSerializer = new JsonSerializer<List<String>>() {
public JsonElement serialize(List<String> src, Type typeOfSrc,
JsonSerializationContext context) {
return new JsonPrimitive(src.get(0) + ";" + src.get(1));
}
};
Gson gson = new GsonBuilder()
.registerTypeAdapter(listOfString, stringListSerializer)
.create();
assertEquals("\"ab;cd\"", gson.toJson(Arrays.asList("ab", "cd"), listOfString));
}
static class HasArrayListField {
ArrayList<Long> longs = new ArrayList<Long>();
}
@SuppressWarnings("rawtypes")
private static int[] toIntArray(Collection collection) {
int[] ints = new int[collection.size()];
int i = 0;
for (Iterator iterator = collection.iterator(); iterator.hasNext(); ++i) {
Object obj = iterator.next();
if (obj instanceof Integer) {
ints[i] = ((Integer)obj).intValue();
} else if (obj instanceof Long) {
ints[i] = ((Long)obj).intValue();
}
}
return ints;
}
private static class ObjectWithWildcardCollection {
private final Collection<? extends BagOfPrimitives> collection;
public ObjectWithWildcardCollection(Collection<? extends BagOfPrimitives> collection) {
this.collection = collection;
}
public Collection<? extends BagOfPrimitives> getCollection() {
return collection;
}
}
private static class Entry {
int value;
Entry(int value) {
this.value = value;
}
}
public void testSetSerialization() {
Set<Entry> set = new HashSet<Entry>();
set.add(new Entry(1));
set.add(new Entry(2));
String json = gson.toJson(set);
assertTrue(json.contains("1"));
assertTrue(json.contains("2"));
}
public void testSetDeserialization() {
String json = "[{value:1},{value:2}]";
Type type = new TypeToken<Set<Entry>>() {}.getType();
Set<Entry> set = gson.fromJson(json, type);
assertEquals(2, set.size());
for (Entry entry : set) {
assertTrue(entry.value == 1 || entry.value == 2);
}
}
private class BigClass { private Map<String, ? extends List<SmallClass>> inBig; }
private class SmallClass { private String inSmall; }
public void testIssue1107() {
String json = "{\n" +
" \"inBig\": {\n" +
" \"key\": [\n" +
" { \"inSmall\": \"hello\" }\n" +
" ]\n" +
" }\n" +
"}";
BigClass bigClass = new Gson().fromJson(json, BigClass.class);
SmallClass small = bigClass.inBig.get("key").get(0);
assertNotNull(small);
assertEquals("hello", small.inSmall);
}
} | // You are a professional Java test case writer, please create a test case named `testIssue1107` for the issue `Gson-1107`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Gson-1107
//
// ## Issue-Title:
// Gson deserializes wildcards to LinkedHashMap
//
// ## Issue-Description:
// This issue is a successor to [#1101](https://github.com/google/gson/issues/1101).
//
//
// Models:
//
//
//
// ```
// // ? extends causes the issue
// class BigClass { Map<String, ? extends List<SmallClass>> inBig; }
//
// class SmallClass { String inSmall; }
// ```
//
// Json:
//
//
//
// ```
// {
// "inBig": {
// "key": [
// { "inSmall": "hello" }
// ]
// }
// }
// ```
//
// Gson call:
//
//
//
// ```
// SmallClass small = new Gson().fromJson(json, BigClass.class).inBig.get("inSmall").get(0);
// ```
//
// This call will fail with a `ClassCastException` exception –
//
// `com.google.gson.internal.LinkedTreeMap cannot be cast to Entry`. If we remove `? extends` then everything works fine.
//
//
//
//
public void testIssue1107() {
| 412 | 18 | 400 | gson/src/test/java/com/google/gson/functional/CollectionTest.java | gson/src/test/java | ```markdown
## Issue-ID: Gson-1107
## Issue-Title:
Gson deserializes wildcards to LinkedHashMap
## Issue-Description:
This issue is a successor to [#1101](https://github.com/google/gson/issues/1101).
Models:
```
// ? extends causes the issue
class BigClass { Map<String, ? extends List<SmallClass>> inBig; }
class SmallClass { String inSmall; }
```
Json:
```
{
"inBig": {
"key": [
{ "inSmall": "hello" }
]
}
}
```
Gson call:
```
SmallClass small = new Gson().fromJson(json, BigClass.class).inBig.get("inSmall").get(0);
```
This call will fail with a `ClassCastException` exception –
`com.google.gson.internal.LinkedTreeMap cannot be cast to Entry`. If we remove `? extends` then everything works fine.
```
You are a professional Java test case writer, please create a test case named `testIssue1107` for the issue `Gson-1107`, utilizing the provided issue report information and the following function signature.
```java
public void testIssue1107() {
```
| 400 | [
"com.google.gson.internal.$Gson$Types"
] | 8c0d9282bfc6aa3fd5c534b6361265cd1d7cbf14fa87d4341015d15e6689579f | public void testIssue1107() | // You are a professional Java test case writer, please create a test case named `testIssue1107` for the issue `Gson-1107`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Gson-1107
//
// ## Issue-Title:
// Gson deserializes wildcards to LinkedHashMap
//
// ## Issue-Description:
// This issue is a successor to [#1101](https://github.com/google/gson/issues/1101).
//
//
// Models:
//
//
//
// ```
// // ? extends causes the issue
// class BigClass { Map<String, ? extends List<SmallClass>> inBig; }
//
// class SmallClass { String inSmall; }
// ```
//
// Json:
//
//
//
// ```
// {
// "inBig": {
// "key": [
// { "inSmall": "hello" }
// ]
// }
// }
// ```
//
// Gson call:
//
//
//
// ```
// SmallClass small = new Gson().fromJson(json, BigClass.class).inBig.get("inSmall").get(0);
// ```
//
// This call will fail with a `ClassCastException` exception –
//
// `com.google.gson.internal.LinkedTreeMap cannot be cast to Entry`. If we remove `? extends` then everything works fine.
//
//
//
//
| Gson | /*
* Copyright (C) 2008 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.gson.functional;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.HashSet;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.PriorityQueue;
import java.util.Queue;
import java.util.Set;
import java.util.Stack;
import java.util.Vector;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonElement;
import com.google.gson.JsonPrimitive;
import com.google.gson.JsonSerializationContext;
import com.google.gson.JsonSerializer;
import com.google.gson.common.MoreAsserts;
import com.google.gson.common.TestTypes.BagOfPrimitives;
import com.google.gson.reflect.TypeToken;
import junit.framework.TestCase;
/**
* Functional tests for Json serialization and deserialization of collections.
*
* @author Inderjeet Singh
* @author Joel Leitch
*/
public class CollectionTest extends TestCase {
private Gson gson;
@Override
protected void setUp() throws Exception {
super.setUp();
gson = new Gson();
}
public void testTopLevelCollectionOfIntegersSerialization() {
Collection<Integer> target = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9);
Type targetType = new TypeToken<Collection<Integer>>() {}.getType();
String json = gson.toJson(target, targetType);
assertEquals("[1,2,3,4,5,6,7,8,9]", json);
}
public void testTopLevelCollectionOfIntegersDeserialization() {
String json = "[0,1,2,3,4,5,6,7,8,9]";
Type collectionType = new TypeToken<Collection<Integer>>() { }.getType();
Collection<Integer> target = gson.fromJson(json, collectionType);
int[] expected = {0, 1, 2, 3, 4, 5, 6, 7, 8, 9};
MoreAsserts.assertEquals(expected, toIntArray(target));
}
public void testTopLevelListOfIntegerCollectionsDeserialization() throws Exception {
String json = "[[1,2,3],[4,5,6],[7,8,9]]";
Type collectionType = new TypeToken<Collection<Collection<Integer>>>() {}.getType();
List<Collection<Integer>> target = gson.fromJson(json, collectionType);
int[][] expected = new int[3][3];
for (int i = 0; i < 3; ++i) {
int start = (3 * i) + 1;
for (int j = 0; j < 3; ++j) {
expected[i][j] = start + j;
}
}
for (int i = 0; i < 3; i++) {
MoreAsserts.assertEquals(expected[i], toIntArray(target.get(i)));
}
}
public void testLinkedListSerialization() {
List<String> list = new LinkedList<String>();
list.add("a1");
list.add("a2");
Type linkedListType = new TypeToken<LinkedList<String>>() {}.getType();
String json = gson.toJson(list, linkedListType);
assertTrue(json.contains("a1"));
assertTrue(json.contains("a2"));
}
public void testLinkedListDeserialization() {
String json = "['a1','a2']";
Type linkedListType = new TypeToken<LinkedList<String>>() {}.getType();
List<String> list = gson.fromJson(json, linkedListType);
assertEquals("a1", list.get(0));
assertEquals("a2", list.get(1));
}
public void testQueueSerialization() {
Queue<String> queue = new LinkedList<String>();
queue.add("a1");
queue.add("a2");
Type queueType = new TypeToken<Queue<String>>() {}.getType();
String json = gson.toJson(queue, queueType);
assertTrue(json.contains("a1"));
assertTrue(json.contains("a2"));
}
public void testQueueDeserialization() {
String json = "['a1','a2']";
Type queueType = new TypeToken<Queue<String>>() {}.getType();
Queue<String> queue = gson.fromJson(json, queueType);
assertEquals("a1", queue.element());
queue.remove();
assertEquals("a2", queue.element());
}
public void testPriorityQueue() throws Exception {
Type type = new TypeToken<PriorityQueue<Integer>>(){}.getType();
PriorityQueue<Integer> queue = gson.fromJson("[10, 20, 22]", type);
assertEquals(3, queue.size());
String json = gson.toJson(queue);
assertEquals(10, queue.remove().intValue());
assertEquals(20, queue.remove().intValue());
assertEquals(22, queue.remove().intValue());
assertEquals("[10,20,22]", json);
}
public void testVector() {
Type type = new TypeToken<Vector<Integer>>(){}.getType();
Vector<Integer> target = gson.fromJson("[10, 20, 31]", type);
assertEquals(3, target.size());
assertEquals(10, target.get(0).intValue());
assertEquals(20, target.get(1).intValue());
assertEquals(31, target.get(2).intValue());
String json = gson.toJson(target);
assertEquals("[10,20,31]", json);
}
public void testStack() {
Type type = new TypeToken<Stack<Integer>>(){}.getType();
Stack<Integer> target = gson.fromJson("[11, 13, 17]", type);
assertEquals(3, target.size());
String json = gson.toJson(target);
assertEquals(17, target.pop().intValue());
assertEquals(13, target.pop().intValue());
assertEquals(11, target.pop().intValue());
assertEquals("[11,13,17]", json);
}
public void testNullsInListSerialization() {
List<String> list = new ArrayList<String>();
list.add("foo");
list.add(null);
list.add("bar");
String expected = "[\"foo\",null,\"bar\"]";
Type typeOfList = new TypeToken<List<String>>() {}.getType();
String json = gson.toJson(list, typeOfList);
assertEquals(expected, json);
}
public void testNullsInListDeserialization() {
List<String> expected = new ArrayList<String>();
expected.add("foo");
expected.add(null);
expected.add("bar");
String json = "[\"foo\",null,\"bar\"]";
Type expectedType = new TypeToken<List<String>>() {}.getType();
List<String> target = gson.fromJson(json, expectedType);
for (int i = 0; i < expected.size(); ++i) {
assertEquals(expected.get(i), target.get(i));
}
}
public void testCollectionOfObjectSerialization() {
List<Object> target = new ArrayList<Object>();
target.add("Hello");
target.add("World");
assertEquals("[\"Hello\",\"World\"]", gson.toJson(target));
Type type = new TypeToken<List<Object>>() {}.getType();
assertEquals("[\"Hello\",\"World\"]", gson.toJson(target, type));
}
public void testCollectionOfObjectWithNullSerialization() {
List<Object> target = new ArrayList<Object>();
target.add("Hello");
target.add(null);
target.add("World");
assertEquals("[\"Hello\",null,\"World\"]", gson.toJson(target));
Type type = new TypeToken<List<Object>>() {}.getType();
assertEquals("[\"Hello\",null,\"World\"]", gson.toJson(target, type));
}
public void testCollectionOfStringsSerialization() {
List<String> target = new ArrayList<String>();
target.add("Hello");
target.add("World");
assertEquals("[\"Hello\",\"World\"]", gson.toJson(target));
}
public void testCollectionOfBagOfPrimitivesSerialization() {
List<BagOfPrimitives> target = new ArrayList<BagOfPrimitives>();
BagOfPrimitives objA = new BagOfPrimitives(3L, 1, true, "blah");
BagOfPrimitives objB = new BagOfPrimitives(2L, 6, false, "blahB");
target.add(objA);
target.add(objB);
String result = gson.toJson(target);
assertTrue(result.startsWith("["));
assertTrue(result.endsWith("]"));
for (BagOfPrimitives obj : target) {
assertTrue(result.contains(obj.getExpectedJson()));
}
}
public void testCollectionOfStringsDeserialization() {
String json = "[\"Hello\",\"World\"]";
Type collectionType = new TypeToken<Collection<String>>() { }.getType();
Collection<String> target = gson.fromJson(json, collectionType);
assertTrue(target.contains("Hello"));
assertTrue(target.contains("World"));
}
public void testRawCollectionOfIntegersSerialization() {
Collection<Integer> target = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9);
assertEquals("[1,2,3,4,5,6,7,8,9]", gson.toJson(target));
}
@SuppressWarnings("rawtypes")
public void testRawCollectionSerialization() {
BagOfPrimitives bag1 = new BagOfPrimitives();
Collection target = Arrays.asList(bag1, bag1);
String json = gson.toJson(target);
assertTrue(json.contains(bag1.getExpectedJson()));
}
@SuppressWarnings("rawtypes")
public void testRawCollectionDeserializationNotAlllowed() {
String json = "[0,1,2,3,4,5,6,7,8,9]";
Collection integers = gson.fromJson(json, Collection.class);
// JsonReader converts numbers to double by default so we need a floating point comparison
assertEquals(Arrays.asList(0.0, 1.0, 2.0, 3.0, 4.0, 5.0, 6.0, 7.0, 8.0, 9.0), integers);
json = "[\"Hello\", \"World\"]";
Collection strings = gson.fromJson(json, Collection.class);
assertTrue(strings.contains("Hello"));
assertTrue(strings.contains("World"));
}
@SuppressWarnings({"rawtypes", "unchecked"})
public void testRawCollectionOfBagOfPrimitivesNotAllowed() {
BagOfPrimitives bag = new BagOfPrimitives(10, 20, false, "stringValue");
String json = '[' + bag.getExpectedJson() + ',' + bag.getExpectedJson() + ']';
Collection target = gson.fromJson(json, Collection.class);
assertEquals(2, target.size());
for (Object bag1 : target) {
// Gson 2.0 converts raw objects into maps
Map<String, Object> values = (Map<String, Object>) bag1;
assertTrue(values.containsValue(10.0));
assertTrue(values.containsValue(20.0));
assertTrue(values.containsValue("stringValue"));
}
}
public void testWildcardPrimitiveCollectionSerilaization() throws Exception {
Collection<? extends Integer> target = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9);
Type collectionType = new TypeToken<Collection<? extends Integer>>() { }.getType();
String json = gson.toJson(target, collectionType);
assertEquals("[1,2,3,4,5,6,7,8,9]", json);
json = gson.toJson(target);
assertEquals("[1,2,3,4,5,6,7,8,9]", json);
}
public void testWildcardPrimitiveCollectionDeserilaization() throws Exception {
String json = "[1,2,3,4,5,6,7,8,9]";
Type collectionType = new TypeToken<Collection<? extends Integer>>() { }.getType();
Collection<? extends Integer> target = gson.fromJson(json, collectionType);
assertEquals(9, target.size());
assertTrue(target.contains(1));
assertTrue(target.contains(9));
}
public void testWildcardCollectionField() throws Exception {
Collection<BagOfPrimitives> collection = new ArrayList<BagOfPrimitives>();
BagOfPrimitives objA = new BagOfPrimitives(3L, 1, true, "blah");
BagOfPrimitives objB = new BagOfPrimitives(2L, 6, false, "blahB");
collection.add(objA);
collection.add(objB);
ObjectWithWildcardCollection target = new ObjectWithWildcardCollection(collection);
String json = gson.toJson(target);
assertTrue(json.contains(objA.getExpectedJson()));
assertTrue(json.contains(objB.getExpectedJson()));
target = gson.fromJson(json, ObjectWithWildcardCollection.class);
Collection<? extends BagOfPrimitives> deserializedCollection = target.getCollection();
assertEquals(2, deserializedCollection.size());
assertTrue(deserializedCollection.contains(objA));
assertTrue(deserializedCollection.contains(objB));
}
public void testFieldIsArrayList() {
HasArrayListField object = new HasArrayListField();
object.longs.add(1L);
object.longs.add(3L);
String json = gson.toJson(object, HasArrayListField.class);
assertEquals("{\"longs\":[1,3]}", json);
HasArrayListField copy = gson.fromJson("{\"longs\":[1,3]}", HasArrayListField.class);
assertEquals(Arrays.asList(1L, 3L), copy.longs);
}
public void testUserCollectionTypeAdapter() {
Type listOfString = new TypeToken<List<String>>() {}.getType();
Object stringListSerializer = new JsonSerializer<List<String>>() {
public JsonElement serialize(List<String> src, Type typeOfSrc,
JsonSerializationContext context) {
return new JsonPrimitive(src.get(0) + ";" + src.get(1));
}
};
Gson gson = new GsonBuilder()
.registerTypeAdapter(listOfString, stringListSerializer)
.create();
assertEquals("\"ab;cd\"", gson.toJson(Arrays.asList("ab", "cd"), listOfString));
}
static class HasArrayListField {
ArrayList<Long> longs = new ArrayList<Long>();
}
@SuppressWarnings("rawtypes")
private static int[] toIntArray(Collection collection) {
int[] ints = new int[collection.size()];
int i = 0;
for (Iterator iterator = collection.iterator(); iterator.hasNext(); ++i) {
Object obj = iterator.next();
if (obj instanceof Integer) {
ints[i] = ((Integer)obj).intValue();
} else if (obj instanceof Long) {
ints[i] = ((Long)obj).intValue();
}
}
return ints;
}
private static class ObjectWithWildcardCollection {
private final Collection<? extends BagOfPrimitives> collection;
public ObjectWithWildcardCollection(Collection<? extends BagOfPrimitives> collection) {
this.collection = collection;
}
public Collection<? extends BagOfPrimitives> getCollection() {
return collection;
}
}
private static class Entry {
int value;
Entry(int value) {
this.value = value;
}
}
public void testSetSerialization() {
Set<Entry> set = new HashSet<Entry>();
set.add(new Entry(1));
set.add(new Entry(2));
String json = gson.toJson(set);
assertTrue(json.contains("1"));
assertTrue(json.contains("2"));
}
public void testSetDeserialization() {
String json = "[{value:1},{value:2}]";
Type type = new TypeToken<Set<Entry>>() {}.getType();
Set<Entry> set = gson.fromJson(json, type);
assertEquals(2, set.size());
for (Entry entry : set) {
assertTrue(entry.value == 1 || entry.value == 2);
}
}
private class BigClass { private Map<String, ? extends List<SmallClass>> inBig; }
private class SmallClass { private String inSmall; }
public void testIssue1107() {
String json = "{\n" +
" \"inBig\": {\n" +
" \"key\": [\n" +
" { \"inSmall\": \"hello\" }\n" +
" ]\n" +
" }\n" +
"}";
BigClass bigClass = new Gson().fromJson(json, BigClass.class);
SmallClass small = bigClass.inBig.get("key").get(0);
assertNotNull(small);
assertEquals("hello", small.inSmall);
}
} |
||
public void testWriteNonAsciiDirectoryNamePosixMode() throws Exception {
String n = "f\u00f6\u00f6/";
TarArchiveEntry t = new TarArchiveEntry(n);
ByteArrayOutputStream bos = new ByteArrayOutputStream();
TarArchiveOutputStream tos = new TarArchiveOutputStream(bos);
tos.setAddPaxHeadersForNonAsciiNames(true);
tos.putArchiveEntry(t);
tos.closeArchiveEntry();
tos.close();
byte[] data = bos.toByteArray();
TarArchiveInputStream tin =
new TarArchiveInputStream(new ByteArrayInputStream(data));
TarArchiveEntry e = tin.getNextTarEntry();
assertEquals(n, e.getName());
assertTrue(e.isDirectory());
tin.close();
} | org.apache.commons.compress.archivers.tar.TarArchiveOutputStreamTest::testWriteNonAsciiDirectoryNamePosixMode | src/test/java/org/apache/commons/compress/archivers/tar/TarArchiveOutputStreamTest.java | 427 | src/test/java/org/apache/commons/compress/archivers/tar/TarArchiveOutputStreamTest.java | testWriteNonAsciiDirectoryNamePosixMode | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package org.apache.commons.compress.archivers.tar;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.util.Calendar;
import java.util.HashMap;
import java.util.Map;
import java.util.TimeZone;
import org.apache.commons.compress.AbstractTestCase;
import org.apache.commons.compress.archivers.ArchiveOutputStream;
import org.apache.commons.compress.archivers.ArchiveStreamFactory;
import org.apache.commons.compress.utils.CharsetNames;
public class TarArchiveOutputStreamTest extends AbstractTestCase {
public void testCount() throws Exception {
File f = File.createTempFile("commons-compress-tarcount", ".tar");
f.deleteOnExit();
FileOutputStream fos = new FileOutputStream(f);
ArchiveOutputStream tarOut = new ArchiveStreamFactory()
.createArchiveOutputStream(ArchiveStreamFactory.TAR, fos);
File file1 = getFile("test1.xml");
TarArchiveEntry sEntry = new TarArchiveEntry(file1);
tarOut.putArchiveEntry(sEntry);
FileInputStream in = new FileInputStream(file1);
byte[] buf = new byte[8192];
int read = 0;
while ((read = in.read(buf)) > 0) {
tarOut.write(buf, 0, read);
}
in.close();
tarOut.closeArchiveEntry();
tarOut.close();
assertEquals(f.length(), tarOut.getBytesWritten());
}
public void testMaxFileSizeError() throws Exception {
TarArchiveEntry t = new TarArchiveEntry("foo");
t.setSize(077777777777L);
TarArchiveOutputStream tos =
new TarArchiveOutputStream(new ByteArrayOutputStream());
tos.putArchiveEntry(t);
t.setSize(0100000000000L);
tos = new TarArchiveOutputStream(new ByteArrayOutputStream());
try {
tos.putArchiveEntry(t);
fail("Should have generated RuntimeException");
} catch (RuntimeException expected) {
}
}
public void testBigNumberStarMode() throws Exception {
TarArchiveEntry t = new TarArchiveEntry("foo");
t.setSize(0100000000000L);
ByteArrayOutputStream bos = new ByteArrayOutputStream();
TarArchiveOutputStream tos = new TarArchiveOutputStream(bos);
tos.setBigNumberMode(TarArchiveOutputStream.BIGNUMBER_STAR);
tos.putArchiveEntry(t);
// make sure header is written to byte array
tos.write(new byte[10 * 1024]);
byte[] data = bos.toByteArray();
assertEquals(0x80,
data[TarConstants.NAMELEN
+ TarConstants.MODELEN
+ TarConstants.UIDLEN
+ TarConstants.GIDLEN] & 0x80);
TarArchiveInputStream tin =
new TarArchiveInputStream(new ByteArrayInputStream(data));
TarArchiveEntry e = tin.getNextTarEntry();
assertEquals(0100000000000L, e.getSize());
tin.close();
// generates IOE because of unclosed entries.
// However we don't really want to create such large entries.
closeQuietly(tos);
}
public void testBigNumberPosixMode() throws Exception {
TarArchiveEntry t = new TarArchiveEntry("foo");
t.setSize(0100000000000L);
ByteArrayOutputStream bos = new ByteArrayOutputStream();
TarArchiveOutputStream tos = new TarArchiveOutputStream(bos);
tos.setBigNumberMode(TarArchiveOutputStream.BIGNUMBER_POSIX);
tos.putArchiveEntry(t);
// make sure header is written to byte array
tos.write(new byte[10 * 1024]);
byte[] data = bos.toByteArray();
assertEquals("00000000000 ",
new String(data,
1024 + TarConstants.NAMELEN
+ TarConstants.MODELEN
+ TarConstants.UIDLEN
+ TarConstants.GIDLEN, 12,
CharsetNames.UTF_8));
TarArchiveInputStream tin =
new TarArchiveInputStream(new ByteArrayInputStream(data));
TarArchiveEntry e = tin.getNextTarEntry();
assertEquals(0100000000000L, e.getSize());
tin.close();
// generates IOE because of unclosed entries.
// However we don't really want to create such large entries.
closeQuietly(tos);
}
public void testWriteSimplePaxHeaders() throws Exception {
Map<String, String> m = new HashMap<String, String>();
m.put("a", "b");
byte[] data = writePaxHeader(m);
assertEquals("00000000006 ",
new String(data, TarConstants.NAMELEN
+ TarConstants.MODELEN
+ TarConstants.UIDLEN
+ TarConstants.GIDLEN, 12,
CharsetNames.UTF_8));
assertEquals("6 a=b\n", new String(data, 512, 6, CharsetNames.UTF_8));
}
public void testPaxHeadersWithLength99() throws Exception {
Map<String, String> m = new HashMap<String, String>();
m.put("a",
"0123456789012345678901234567890123456789"
+ "01234567890123456789012345678901234567890123456789"
+ "012");
byte[] data = writePaxHeader(m);
assertEquals("00000000143 ",
new String(data, TarConstants.NAMELEN
+ TarConstants.MODELEN
+ TarConstants.UIDLEN
+ TarConstants.GIDLEN, 12,
CharsetNames.UTF_8));
assertEquals("99 a=0123456789012345678901234567890123456789"
+ "01234567890123456789012345678901234567890123456789"
+ "012\n", new String(data, 512, 99, CharsetNames.UTF_8));
}
public void testPaxHeadersWithLength101() throws Exception {
Map<String, String> m = new HashMap<String, String>();
m.put("a",
"0123456789012345678901234567890123456789"
+ "01234567890123456789012345678901234567890123456789"
+ "0123");
byte[] data = writePaxHeader(m);
assertEquals("00000000145 ",
new String(data, TarConstants.NAMELEN
+ TarConstants.MODELEN
+ TarConstants.UIDLEN
+ TarConstants.GIDLEN, 12,
CharsetNames.UTF_8));
assertEquals("101 a=0123456789012345678901234567890123456789"
+ "01234567890123456789012345678901234567890123456789"
+ "0123\n", new String(data, 512, 101, CharsetNames.UTF_8));
}
private byte[] writePaxHeader(Map<String, String> m) throws Exception {
ByteArrayOutputStream bos = new ByteArrayOutputStream();
TarArchiveOutputStream tos = new TarArchiveOutputStream(bos, "ASCII");
tos.writePaxHeaders("foo", m);
// add a dummy entry so data gets written
TarArchiveEntry t = new TarArchiveEntry("foo");
t.setSize(10 * 1024);
tos.putArchiveEntry(t);
tos.write(new byte[10 * 1024]);
tos.closeArchiveEntry();
tos.close();
return bos.toByteArray();
}
public void testWriteLongFileNamePosixMode() throws Exception {
String n = "01234567890123456789012345678901234567890123456789"
+ "01234567890123456789012345678901234567890123456789"
+ "01234567890123456789012345678901234567890123456789";
TarArchiveEntry t =
new TarArchiveEntry(n);
t.setSize(10 * 1024);
ByteArrayOutputStream bos = new ByteArrayOutputStream();
TarArchiveOutputStream tos = new TarArchiveOutputStream(bos, "ASCII");
tos.setLongFileMode(TarArchiveOutputStream.LONGFILE_POSIX);
tos.putArchiveEntry(t);
tos.write(new byte[10 * 1024]);
tos.closeArchiveEntry();
byte[] data = bos.toByteArray();
assertEquals("160 path=" + n + "\n",
new String(data, 512, 160, CharsetNames.UTF_8));
TarArchiveInputStream tin =
new TarArchiveInputStream(new ByteArrayInputStream(data));
TarArchiveEntry e = tin.getNextTarEntry();
assertEquals(n, e.getName());
tin.close();
tos.close();
}
public void testOldEntryStarMode() throws Exception {
TarArchiveEntry t = new TarArchiveEntry("foo");
t.setSize(Integer.MAX_VALUE);
t.setModTime(-1000);
ByteArrayOutputStream bos = new ByteArrayOutputStream();
TarArchiveOutputStream tos = new TarArchiveOutputStream(bos);
tos.setBigNumberMode(TarArchiveOutputStream.BIGNUMBER_STAR);
tos.putArchiveEntry(t);
// make sure header is written to byte array
tos.write(new byte[10 * 1024]);
byte[] data = bos.toByteArray();
assertEquals((byte) 0xff,
data[TarConstants.NAMELEN
+ TarConstants.MODELEN
+ TarConstants.UIDLEN
+ TarConstants.GIDLEN
+ TarConstants.SIZELEN]);
TarArchiveInputStream tin =
new TarArchiveInputStream(new ByteArrayInputStream(data));
TarArchiveEntry e = tin.getNextTarEntry();
Calendar cal = Calendar.getInstance(TimeZone.getTimeZone("GMT"));
cal.set(1969, 11, 31, 23, 59, 59);
cal.set(Calendar.MILLISECOND, 0);
assertEquals(cal.getTime(), e.getLastModifiedDate());
tin.close();
// generates IOE because of unclosed entries.
// However we don't really want to create such large entries.
closeQuietly(tos);
}
public void testOldEntryPosixMode() throws Exception {
TarArchiveEntry t = new TarArchiveEntry("foo");
t.setSize(Integer.MAX_VALUE);
t.setModTime(-1000);
ByteArrayOutputStream bos = new ByteArrayOutputStream();
TarArchiveOutputStream tos = new TarArchiveOutputStream(bos);
tos.setBigNumberMode(TarArchiveOutputStream.BIGNUMBER_POSIX);
tos.putArchiveEntry(t);
// make sure header is written to byte array
tos.write(new byte[10 * 1024]);
byte[] data = bos.toByteArray();
assertEquals("00000000000 ",
new String(data,
1024 + TarConstants.NAMELEN
+ TarConstants.MODELEN
+ TarConstants.UIDLEN
+ TarConstants.GIDLEN
+ TarConstants.SIZELEN, 12,
CharsetNames.UTF_8));
TarArchiveInputStream tin =
new TarArchiveInputStream(new ByteArrayInputStream(data));
TarArchiveEntry e = tin.getNextTarEntry();
Calendar cal = Calendar.getInstance(TimeZone.getTimeZone("GMT"));
cal.set(1969, 11, 31, 23, 59, 59);
cal.set(Calendar.MILLISECOND, 0);
assertEquals(cal.getTime(), e.getLastModifiedDate());
tin.close();
// generates IOE because of unclosed entries.
// However we don't really want to create such large entries.
closeQuietly(tos);
}
public void testOldEntryError() throws Exception {
TarArchiveEntry t = new TarArchiveEntry("foo");
t.setSize(Integer.MAX_VALUE);
t.setModTime(-1000);
TarArchiveOutputStream tos =
new TarArchiveOutputStream(new ByteArrayOutputStream());
try {
tos.putArchiveEntry(t);
fail("Should have generated RuntimeException");
} catch (RuntimeException expected) {
}
tos.close();
}
public void testWriteNonAsciiPathNamePaxHeader() throws Exception {
String n = "\u00e4";
TarArchiveEntry t = new TarArchiveEntry(n);
t.setSize(10 * 1024);
ByteArrayOutputStream bos = new ByteArrayOutputStream();
TarArchiveOutputStream tos = new TarArchiveOutputStream(bos);
tos.setAddPaxHeadersForNonAsciiNames(true);
tos.putArchiveEntry(t);
tos.write(new byte[10 * 1024]);
tos.closeArchiveEntry();
tos.close();
byte[] data = bos.toByteArray();
assertEquals("11 path=" + n + "\n",
new String(data, 512, 11, CharsetNames.UTF_8));
TarArchiveInputStream tin =
new TarArchiveInputStream(new ByteArrayInputStream(data));
TarArchiveEntry e = tin.getNextTarEntry();
assertEquals(n, e.getName());
tin.close();
}
public void testWriteNonAsciiLinkPathNamePaxHeader() throws Exception {
String n = "\u00e4";
TarArchiveEntry t = new TarArchiveEntry("a", TarConstants.LF_LINK);
t.setSize(10 * 1024);
t.setLinkName(n);
ByteArrayOutputStream bos = new ByteArrayOutputStream();
TarArchiveOutputStream tos = new TarArchiveOutputStream(bos);
tos.setAddPaxHeadersForNonAsciiNames(true);
tos.putArchiveEntry(t);
tos.write(new byte[10 * 1024]);
tos.closeArchiveEntry();
tos.close();
byte[] data = bos.toByteArray();
assertEquals("15 linkpath=" + n + "\n",
new String(data, 512, 15, CharsetNames.UTF_8));
TarArchiveInputStream tin =
new TarArchiveInputStream(new ByteArrayInputStream(data));
TarArchiveEntry e = tin.getNextTarEntry();
assertEquals(n, e.getLinkName());
tin.close();
}
/**
* @see https://issues.apache.org/jira/browse/COMPRESS-200
*/
public void testRoundtripWith67CharFileNameGnu() throws Exception {
testRoundtripWith67CharFileName(TarArchiveOutputStream.LONGFILE_GNU);
}
/**
* @see https://issues.apache.org/jira/browse/COMPRESS-200
*/
public void testRoundtripWith67CharFileNamePosix() throws Exception {
testRoundtripWith67CharFileName(TarArchiveOutputStream.LONGFILE_POSIX);
}
private void testRoundtripWith67CharFileName(int mode) throws Exception {
String n = "AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA"
+ "AAAAAAA";
assertEquals(67, n.length());
TarArchiveEntry t = new TarArchiveEntry(n);
t.setSize(10 * 1024);
ByteArrayOutputStream bos = new ByteArrayOutputStream();
TarArchiveOutputStream tos = new TarArchiveOutputStream(bos, "ASCII");
tos.setLongFileMode(mode);
tos.putArchiveEntry(t);
tos.write(new byte[10 * 1024]);
tos.closeArchiveEntry();
tos.close();
byte[] data = bos.toByteArray();
TarArchiveInputStream tin =
new TarArchiveInputStream(new ByteArrayInputStream(data));
TarArchiveEntry e = tin.getNextTarEntry();
assertEquals(n, e.getName());
tin.close();
}
/**
* @see https://issues.apache.org/jira/browse/COMPRESS-203
*/
public void testWriteLongDirectoryNameGnuMode() throws Exception {
testWriteLongDirectoryName(TarArchiveOutputStream.LONGFILE_GNU);
}
/**
* @see https://issues.apache.org/jira/browse/COMPRESS-203
*/
public void testWriteLongDirectoryNamePosixMode() throws Exception {
testWriteLongDirectoryName(TarArchiveOutputStream.LONGFILE_POSIX);
}
private void testWriteLongDirectoryName(int mode) throws Exception {
String n = "01234567890123456789012345678901234567890123456789"
+ "01234567890123456789012345678901234567890123456789"
+ "01234567890123456789012345678901234567890123456789/";
TarArchiveEntry t = new TarArchiveEntry(n);
ByteArrayOutputStream bos = new ByteArrayOutputStream();
TarArchiveOutputStream tos = new TarArchiveOutputStream(bos, "ASCII");
tos.setLongFileMode(mode);
tos.putArchiveEntry(t);
tos.closeArchiveEntry();
tos.close();
byte[] data = bos.toByteArray();
TarArchiveInputStream tin =
new TarArchiveInputStream(new ByteArrayInputStream(data));
TarArchiveEntry e = tin.getNextTarEntry();
assertEquals(n, e.getName());
assertTrue(e.isDirectory());
tin.close();
}
/**
* @see https://issues.apache.org/jira/browse/COMPRESS-203
*/
public void testWriteNonAsciiDirectoryNamePosixMode() throws Exception {
String n = "f\u00f6\u00f6/";
TarArchiveEntry t = new TarArchiveEntry(n);
ByteArrayOutputStream bos = new ByteArrayOutputStream();
TarArchiveOutputStream tos = new TarArchiveOutputStream(bos);
tos.setAddPaxHeadersForNonAsciiNames(true);
tos.putArchiveEntry(t);
tos.closeArchiveEntry();
tos.close();
byte[] data = bos.toByteArray();
TarArchiveInputStream tin =
new TarArchiveInputStream(new ByteArrayInputStream(data));
TarArchiveEntry e = tin.getNextTarEntry();
assertEquals(n, e.getName());
assertTrue(e.isDirectory());
tin.close();
}
} | // You are a professional Java test case writer, please create a test case named `testWriteNonAsciiDirectoryNamePosixMode` for the issue `Compress-COMPRESS-203`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Compress-COMPRESS-203
//
// ## Issue-Title:
// Long directory names can not be stored in a tar archive because of error when writing PAX headers
//
// ## Issue-Description:
//
// Trying to add a directory to the TAR Archive that has a name longer than 100 bytes generates an exception with a stack trace similar to the following:
//
//
//
//
// ```
// java.io.IOException: request to write '114' bytes exceeds size in header of '0' bytes for entry './PaxHeaders.X/layers/openstreetmap__osm.disy.net/.tiles/1.0.0/openstreetmap__osm.disy.net/default/'
//
// at org.apache.commons.compress.archivers.tar.TarArchiveOutputStream.write(TarArchiveOutputStream.java:385)
//
// at java.io.OutputStream.write(Unknown Source)
//
// at org.apache.commons.compress.archivers.tar.TarArchiveOutputStream.writePaxHeaders(TarArchiveOutputStream.java:485)
//
// at org.apache.commons.compress.archivers.tar.TarArchiveOutputStream.putArchiveEntry(TarArchiveOutputStream.java:312)
//
// at net.disy.lib.io.tar.TarUtilities.addFile(TarUtilities.java:116)
//
// at net.disy.lib.io.tar.TarUtilities.addDirectory(TarUtilities.java:158)
//
// at net.disy.lib.io.tar.TarUtilities.addDirectory(TarUtilities.java:162)
//
// at net.disy.lib.io.tar.TarUtilities.addDirectory(TarUtilities.java:162)
//
// at net.disy.lib.io.tar.TarUtilities.addDirectory(TarUtilities.java:162)
//
// at net.disy.lib.io.tar.TarUtilities.addDirectory(TarUtilities.java:162)
//
// at net.disy.lib.io.tar.TarUtilities.addDirectory(TarUtilities.java:162)
//
// at net.disy.lib.io.tar.TarUtilities.addDirectory(TarUtilities.java:162)
//
// at net.disy.lib.io.tar.TarUtilities.addDirectory(TarUtilities.java:162)
//
// at net.disy.lib.io.tar.TarUtilities.addDirectory(TarUtilities.java:162)
//
// at net.disy.lib.io.tar.TarUtilities.addDirectory(TarUtilities.java:162)
//
// at net.disy.lib.io.tar.TarUtilities.tar(TarUtilities.java:77)
//
// at net.disy.lib.io.tar.TarUtilities.tar(TarUtilities.java:42)
//
// at net.disy.gisterm.tilecacheset.export.TileCacheSetExporter.tarTreeStructure(TileCacheSetExporter.java:262)
//
// at net.disy.gisterm.tilecacheset.export.TileCacheSetExporter.export(TileCacheSetExporter.java:111)
//
// at net.disy.gisterm.tilecacheset.desktop.controller.ExportController$1.run(ExportController.java:81)
//
// ... 2 more
//
// ```
//
//
// Informal source code investigation points to the problem being that for directory entries the code assumes that the length is 0 in putArchive
public void testWriteNonAsciiDirectoryNamePosixMode() throws Exception {
| 427 | /**
* @see https://issues.apache.org/jira/browse/COMPRESS-203
*/ | 18 | 411 | src/test/java/org/apache/commons/compress/archivers/tar/TarArchiveOutputStreamTest.java | src/test/java | ```markdown
## Issue-ID: Compress-COMPRESS-203
## Issue-Title:
Long directory names can not be stored in a tar archive because of error when writing PAX headers
## Issue-Description:
Trying to add a directory to the TAR Archive that has a name longer than 100 bytes generates an exception with a stack trace similar to the following:
```
java.io.IOException: request to write '114' bytes exceeds size in header of '0' bytes for entry './PaxHeaders.X/layers/openstreetmap__osm.disy.net/.tiles/1.0.0/openstreetmap__osm.disy.net/default/'
at org.apache.commons.compress.archivers.tar.TarArchiveOutputStream.write(TarArchiveOutputStream.java:385)
at java.io.OutputStream.write(Unknown Source)
at org.apache.commons.compress.archivers.tar.TarArchiveOutputStream.writePaxHeaders(TarArchiveOutputStream.java:485)
at org.apache.commons.compress.archivers.tar.TarArchiveOutputStream.putArchiveEntry(TarArchiveOutputStream.java:312)
at net.disy.lib.io.tar.TarUtilities.addFile(TarUtilities.java:116)
at net.disy.lib.io.tar.TarUtilities.addDirectory(TarUtilities.java:158)
at net.disy.lib.io.tar.TarUtilities.addDirectory(TarUtilities.java:162)
at net.disy.lib.io.tar.TarUtilities.addDirectory(TarUtilities.java:162)
at net.disy.lib.io.tar.TarUtilities.addDirectory(TarUtilities.java:162)
at net.disy.lib.io.tar.TarUtilities.addDirectory(TarUtilities.java:162)
at net.disy.lib.io.tar.TarUtilities.addDirectory(TarUtilities.java:162)
at net.disy.lib.io.tar.TarUtilities.addDirectory(TarUtilities.java:162)
at net.disy.lib.io.tar.TarUtilities.addDirectory(TarUtilities.java:162)
at net.disy.lib.io.tar.TarUtilities.addDirectory(TarUtilities.java:162)
at net.disy.lib.io.tar.TarUtilities.addDirectory(TarUtilities.java:162)
at net.disy.lib.io.tar.TarUtilities.tar(TarUtilities.java:77)
at net.disy.lib.io.tar.TarUtilities.tar(TarUtilities.java:42)
at net.disy.gisterm.tilecacheset.export.TileCacheSetExporter.tarTreeStructure(TileCacheSetExporter.java:262)
at net.disy.gisterm.tilecacheset.export.TileCacheSetExporter.export(TileCacheSetExporter.java:111)
at net.disy.gisterm.tilecacheset.desktop.controller.ExportController$1.run(ExportController.java:81)
... 2 more
```
Informal source code investigation points to the problem being that for directory entries the code assumes that the length is 0 in putArchive
```
You are a professional Java test case writer, please create a test case named `testWriteNonAsciiDirectoryNamePosixMode` for the issue `Compress-COMPRESS-203`, utilizing the provided issue report information and the following function signature.
```java
public void testWriteNonAsciiDirectoryNamePosixMode() throws Exception {
```
| 411 | [
"org.apache.commons.compress.archivers.tar.TarArchiveOutputStream"
] | 8c7b1b8f75d0e6749f1b39dc530151814d2456dfaeb47f75d84ae8d6d029b182 | public void testWriteNonAsciiDirectoryNamePosixMode() throws Exception | // You are a professional Java test case writer, please create a test case named `testWriteNonAsciiDirectoryNamePosixMode` for the issue `Compress-COMPRESS-203`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Compress-COMPRESS-203
//
// ## Issue-Title:
// Long directory names can not be stored in a tar archive because of error when writing PAX headers
//
// ## Issue-Description:
//
// Trying to add a directory to the TAR Archive that has a name longer than 100 bytes generates an exception with a stack trace similar to the following:
//
//
//
//
// ```
// java.io.IOException: request to write '114' bytes exceeds size in header of '0' bytes for entry './PaxHeaders.X/layers/openstreetmap__osm.disy.net/.tiles/1.0.0/openstreetmap__osm.disy.net/default/'
//
// at org.apache.commons.compress.archivers.tar.TarArchiveOutputStream.write(TarArchiveOutputStream.java:385)
//
// at java.io.OutputStream.write(Unknown Source)
//
// at org.apache.commons.compress.archivers.tar.TarArchiveOutputStream.writePaxHeaders(TarArchiveOutputStream.java:485)
//
// at org.apache.commons.compress.archivers.tar.TarArchiveOutputStream.putArchiveEntry(TarArchiveOutputStream.java:312)
//
// at net.disy.lib.io.tar.TarUtilities.addFile(TarUtilities.java:116)
//
// at net.disy.lib.io.tar.TarUtilities.addDirectory(TarUtilities.java:158)
//
// at net.disy.lib.io.tar.TarUtilities.addDirectory(TarUtilities.java:162)
//
// at net.disy.lib.io.tar.TarUtilities.addDirectory(TarUtilities.java:162)
//
// at net.disy.lib.io.tar.TarUtilities.addDirectory(TarUtilities.java:162)
//
// at net.disy.lib.io.tar.TarUtilities.addDirectory(TarUtilities.java:162)
//
// at net.disy.lib.io.tar.TarUtilities.addDirectory(TarUtilities.java:162)
//
// at net.disy.lib.io.tar.TarUtilities.addDirectory(TarUtilities.java:162)
//
// at net.disy.lib.io.tar.TarUtilities.addDirectory(TarUtilities.java:162)
//
// at net.disy.lib.io.tar.TarUtilities.addDirectory(TarUtilities.java:162)
//
// at net.disy.lib.io.tar.TarUtilities.addDirectory(TarUtilities.java:162)
//
// at net.disy.lib.io.tar.TarUtilities.tar(TarUtilities.java:77)
//
// at net.disy.lib.io.tar.TarUtilities.tar(TarUtilities.java:42)
//
// at net.disy.gisterm.tilecacheset.export.TileCacheSetExporter.tarTreeStructure(TileCacheSetExporter.java:262)
//
// at net.disy.gisterm.tilecacheset.export.TileCacheSetExporter.export(TileCacheSetExporter.java:111)
//
// at net.disy.gisterm.tilecacheset.desktop.controller.ExportController$1.run(ExportController.java:81)
//
// ... 2 more
//
// ```
//
//
// Informal source code investigation points to the problem being that for directory entries the code assumes that the length is 0 in putArchive
| Compress | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package org.apache.commons.compress.archivers.tar;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.util.Calendar;
import java.util.HashMap;
import java.util.Map;
import java.util.TimeZone;
import org.apache.commons.compress.AbstractTestCase;
import org.apache.commons.compress.archivers.ArchiveOutputStream;
import org.apache.commons.compress.archivers.ArchiveStreamFactory;
import org.apache.commons.compress.utils.CharsetNames;
public class TarArchiveOutputStreamTest extends AbstractTestCase {
public void testCount() throws Exception {
File f = File.createTempFile("commons-compress-tarcount", ".tar");
f.deleteOnExit();
FileOutputStream fos = new FileOutputStream(f);
ArchiveOutputStream tarOut = new ArchiveStreamFactory()
.createArchiveOutputStream(ArchiveStreamFactory.TAR, fos);
File file1 = getFile("test1.xml");
TarArchiveEntry sEntry = new TarArchiveEntry(file1);
tarOut.putArchiveEntry(sEntry);
FileInputStream in = new FileInputStream(file1);
byte[] buf = new byte[8192];
int read = 0;
while ((read = in.read(buf)) > 0) {
tarOut.write(buf, 0, read);
}
in.close();
tarOut.closeArchiveEntry();
tarOut.close();
assertEquals(f.length(), tarOut.getBytesWritten());
}
public void testMaxFileSizeError() throws Exception {
TarArchiveEntry t = new TarArchiveEntry("foo");
t.setSize(077777777777L);
TarArchiveOutputStream tos =
new TarArchiveOutputStream(new ByteArrayOutputStream());
tos.putArchiveEntry(t);
t.setSize(0100000000000L);
tos = new TarArchiveOutputStream(new ByteArrayOutputStream());
try {
tos.putArchiveEntry(t);
fail("Should have generated RuntimeException");
} catch (RuntimeException expected) {
}
}
public void testBigNumberStarMode() throws Exception {
TarArchiveEntry t = new TarArchiveEntry("foo");
t.setSize(0100000000000L);
ByteArrayOutputStream bos = new ByteArrayOutputStream();
TarArchiveOutputStream tos = new TarArchiveOutputStream(bos);
tos.setBigNumberMode(TarArchiveOutputStream.BIGNUMBER_STAR);
tos.putArchiveEntry(t);
// make sure header is written to byte array
tos.write(new byte[10 * 1024]);
byte[] data = bos.toByteArray();
assertEquals(0x80,
data[TarConstants.NAMELEN
+ TarConstants.MODELEN
+ TarConstants.UIDLEN
+ TarConstants.GIDLEN] & 0x80);
TarArchiveInputStream tin =
new TarArchiveInputStream(new ByteArrayInputStream(data));
TarArchiveEntry e = tin.getNextTarEntry();
assertEquals(0100000000000L, e.getSize());
tin.close();
// generates IOE because of unclosed entries.
// However we don't really want to create such large entries.
closeQuietly(tos);
}
public void testBigNumberPosixMode() throws Exception {
TarArchiveEntry t = new TarArchiveEntry("foo");
t.setSize(0100000000000L);
ByteArrayOutputStream bos = new ByteArrayOutputStream();
TarArchiveOutputStream tos = new TarArchiveOutputStream(bos);
tos.setBigNumberMode(TarArchiveOutputStream.BIGNUMBER_POSIX);
tos.putArchiveEntry(t);
// make sure header is written to byte array
tos.write(new byte[10 * 1024]);
byte[] data = bos.toByteArray();
assertEquals("00000000000 ",
new String(data,
1024 + TarConstants.NAMELEN
+ TarConstants.MODELEN
+ TarConstants.UIDLEN
+ TarConstants.GIDLEN, 12,
CharsetNames.UTF_8));
TarArchiveInputStream tin =
new TarArchiveInputStream(new ByteArrayInputStream(data));
TarArchiveEntry e = tin.getNextTarEntry();
assertEquals(0100000000000L, e.getSize());
tin.close();
// generates IOE because of unclosed entries.
// However we don't really want to create such large entries.
closeQuietly(tos);
}
public void testWriteSimplePaxHeaders() throws Exception {
Map<String, String> m = new HashMap<String, String>();
m.put("a", "b");
byte[] data = writePaxHeader(m);
assertEquals("00000000006 ",
new String(data, TarConstants.NAMELEN
+ TarConstants.MODELEN
+ TarConstants.UIDLEN
+ TarConstants.GIDLEN, 12,
CharsetNames.UTF_8));
assertEquals("6 a=b\n", new String(data, 512, 6, CharsetNames.UTF_8));
}
public void testPaxHeadersWithLength99() throws Exception {
Map<String, String> m = new HashMap<String, String>();
m.put("a",
"0123456789012345678901234567890123456789"
+ "01234567890123456789012345678901234567890123456789"
+ "012");
byte[] data = writePaxHeader(m);
assertEquals("00000000143 ",
new String(data, TarConstants.NAMELEN
+ TarConstants.MODELEN
+ TarConstants.UIDLEN
+ TarConstants.GIDLEN, 12,
CharsetNames.UTF_8));
assertEquals("99 a=0123456789012345678901234567890123456789"
+ "01234567890123456789012345678901234567890123456789"
+ "012\n", new String(data, 512, 99, CharsetNames.UTF_8));
}
public void testPaxHeadersWithLength101() throws Exception {
Map<String, String> m = new HashMap<String, String>();
m.put("a",
"0123456789012345678901234567890123456789"
+ "01234567890123456789012345678901234567890123456789"
+ "0123");
byte[] data = writePaxHeader(m);
assertEquals("00000000145 ",
new String(data, TarConstants.NAMELEN
+ TarConstants.MODELEN
+ TarConstants.UIDLEN
+ TarConstants.GIDLEN, 12,
CharsetNames.UTF_8));
assertEquals("101 a=0123456789012345678901234567890123456789"
+ "01234567890123456789012345678901234567890123456789"
+ "0123\n", new String(data, 512, 101, CharsetNames.UTF_8));
}
private byte[] writePaxHeader(Map<String, String> m) throws Exception {
ByteArrayOutputStream bos = new ByteArrayOutputStream();
TarArchiveOutputStream tos = new TarArchiveOutputStream(bos, "ASCII");
tos.writePaxHeaders("foo", m);
// add a dummy entry so data gets written
TarArchiveEntry t = new TarArchiveEntry("foo");
t.setSize(10 * 1024);
tos.putArchiveEntry(t);
tos.write(new byte[10 * 1024]);
tos.closeArchiveEntry();
tos.close();
return bos.toByteArray();
}
public void testWriteLongFileNamePosixMode() throws Exception {
String n = "01234567890123456789012345678901234567890123456789"
+ "01234567890123456789012345678901234567890123456789"
+ "01234567890123456789012345678901234567890123456789";
TarArchiveEntry t =
new TarArchiveEntry(n);
t.setSize(10 * 1024);
ByteArrayOutputStream bos = new ByteArrayOutputStream();
TarArchiveOutputStream tos = new TarArchiveOutputStream(bos, "ASCII");
tos.setLongFileMode(TarArchiveOutputStream.LONGFILE_POSIX);
tos.putArchiveEntry(t);
tos.write(new byte[10 * 1024]);
tos.closeArchiveEntry();
byte[] data = bos.toByteArray();
assertEquals("160 path=" + n + "\n",
new String(data, 512, 160, CharsetNames.UTF_8));
TarArchiveInputStream tin =
new TarArchiveInputStream(new ByteArrayInputStream(data));
TarArchiveEntry e = tin.getNextTarEntry();
assertEquals(n, e.getName());
tin.close();
tos.close();
}
public void testOldEntryStarMode() throws Exception {
TarArchiveEntry t = new TarArchiveEntry("foo");
t.setSize(Integer.MAX_VALUE);
t.setModTime(-1000);
ByteArrayOutputStream bos = new ByteArrayOutputStream();
TarArchiveOutputStream tos = new TarArchiveOutputStream(bos);
tos.setBigNumberMode(TarArchiveOutputStream.BIGNUMBER_STAR);
tos.putArchiveEntry(t);
// make sure header is written to byte array
tos.write(new byte[10 * 1024]);
byte[] data = bos.toByteArray();
assertEquals((byte) 0xff,
data[TarConstants.NAMELEN
+ TarConstants.MODELEN
+ TarConstants.UIDLEN
+ TarConstants.GIDLEN
+ TarConstants.SIZELEN]);
TarArchiveInputStream tin =
new TarArchiveInputStream(new ByteArrayInputStream(data));
TarArchiveEntry e = tin.getNextTarEntry();
Calendar cal = Calendar.getInstance(TimeZone.getTimeZone("GMT"));
cal.set(1969, 11, 31, 23, 59, 59);
cal.set(Calendar.MILLISECOND, 0);
assertEquals(cal.getTime(), e.getLastModifiedDate());
tin.close();
// generates IOE because of unclosed entries.
// However we don't really want to create such large entries.
closeQuietly(tos);
}
public void testOldEntryPosixMode() throws Exception {
TarArchiveEntry t = new TarArchiveEntry("foo");
t.setSize(Integer.MAX_VALUE);
t.setModTime(-1000);
ByteArrayOutputStream bos = new ByteArrayOutputStream();
TarArchiveOutputStream tos = new TarArchiveOutputStream(bos);
tos.setBigNumberMode(TarArchiveOutputStream.BIGNUMBER_POSIX);
tos.putArchiveEntry(t);
// make sure header is written to byte array
tos.write(new byte[10 * 1024]);
byte[] data = bos.toByteArray();
assertEquals("00000000000 ",
new String(data,
1024 + TarConstants.NAMELEN
+ TarConstants.MODELEN
+ TarConstants.UIDLEN
+ TarConstants.GIDLEN
+ TarConstants.SIZELEN, 12,
CharsetNames.UTF_8));
TarArchiveInputStream tin =
new TarArchiveInputStream(new ByteArrayInputStream(data));
TarArchiveEntry e = tin.getNextTarEntry();
Calendar cal = Calendar.getInstance(TimeZone.getTimeZone("GMT"));
cal.set(1969, 11, 31, 23, 59, 59);
cal.set(Calendar.MILLISECOND, 0);
assertEquals(cal.getTime(), e.getLastModifiedDate());
tin.close();
// generates IOE because of unclosed entries.
// However we don't really want to create such large entries.
closeQuietly(tos);
}
public void testOldEntryError() throws Exception {
TarArchiveEntry t = new TarArchiveEntry("foo");
t.setSize(Integer.MAX_VALUE);
t.setModTime(-1000);
TarArchiveOutputStream tos =
new TarArchiveOutputStream(new ByteArrayOutputStream());
try {
tos.putArchiveEntry(t);
fail("Should have generated RuntimeException");
} catch (RuntimeException expected) {
}
tos.close();
}
public void testWriteNonAsciiPathNamePaxHeader() throws Exception {
String n = "\u00e4";
TarArchiveEntry t = new TarArchiveEntry(n);
t.setSize(10 * 1024);
ByteArrayOutputStream bos = new ByteArrayOutputStream();
TarArchiveOutputStream tos = new TarArchiveOutputStream(bos);
tos.setAddPaxHeadersForNonAsciiNames(true);
tos.putArchiveEntry(t);
tos.write(new byte[10 * 1024]);
tos.closeArchiveEntry();
tos.close();
byte[] data = bos.toByteArray();
assertEquals("11 path=" + n + "\n",
new String(data, 512, 11, CharsetNames.UTF_8));
TarArchiveInputStream tin =
new TarArchiveInputStream(new ByteArrayInputStream(data));
TarArchiveEntry e = tin.getNextTarEntry();
assertEquals(n, e.getName());
tin.close();
}
public void testWriteNonAsciiLinkPathNamePaxHeader() throws Exception {
String n = "\u00e4";
TarArchiveEntry t = new TarArchiveEntry("a", TarConstants.LF_LINK);
t.setSize(10 * 1024);
t.setLinkName(n);
ByteArrayOutputStream bos = new ByteArrayOutputStream();
TarArchiveOutputStream tos = new TarArchiveOutputStream(bos);
tos.setAddPaxHeadersForNonAsciiNames(true);
tos.putArchiveEntry(t);
tos.write(new byte[10 * 1024]);
tos.closeArchiveEntry();
tos.close();
byte[] data = bos.toByteArray();
assertEquals("15 linkpath=" + n + "\n",
new String(data, 512, 15, CharsetNames.UTF_8));
TarArchiveInputStream tin =
new TarArchiveInputStream(new ByteArrayInputStream(data));
TarArchiveEntry e = tin.getNextTarEntry();
assertEquals(n, e.getLinkName());
tin.close();
}
/**
* @see https://issues.apache.org/jira/browse/COMPRESS-200
*/
public void testRoundtripWith67CharFileNameGnu() throws Exception {
testRoundtripWith67CharFileName(TarArchiveOutputStream.LONGFILE_GNU);
}
/**
* @see https://issues.apache.org/jira/browse/COMPRESS-200
*/
public void testRoundtripWith67CharFileNamePosix() throws Exception {
testRoundtripWith67CharFileName(TarArchiveOutputStream.LONGFILE_POSIX);
}
private void testRoundtripWith67CharFileName(int mode) throws Exception {
String n = "AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA"
+ "AAAAAAA";
assertEquals(67, n.length());
TarArchiveEntry t = new TarArchiveEntry(n);
t.setSize(10 * 1024);
ByteArrayOutputStream bos = new ByteArrayOutputStream();
TarArchiveOutputStream tos = new TarArchiveOutputStream(bos, "ASCII");
tos.setLongFileMode(mode);
tos.putArchiveEntry(t);
tos.write(new byte[10 * 1024]);
tos.closeArchiveEntry();
tos.close();
byte[] data = bos.toByteArray();
TarArchiveInputStream tin =
new TarArchiveInputStream(new ByteArrayInputStream(data));
TarArchiveEntry e = tin.getNextTarEntry();
assertEquals(n, e.getName());
tin.close();
}
/**
* @see https://issues.apache.org/jira/browse/COMPRESS-203
*/
public void testWriteLongDirectoryNameGnuMode() throws Exception {
testWriteLongDirectoryName(TarArchiveOutputStream.LONGFILE_GNU);
}
/**
* @see https://issues.apache.org/jira/browse/COMPRESS-203
*/
public void testWriteLongDirectoryNamePosixMode() throws Exception {
testWriteLongDirectoryName(TarArchiveOutputStream.LONGFILE_POSIX);
}
private void testWriteLongDirectoryName(int mode) throws Exception {
String n = "01234567890123456789012345678901234567890123456789"
+ "01234567890123456789012345678901234567890123456789"
+ "01234567890123456789012345678901234567890123456789/";
TarArchiveEntry t = new TarArchiveEntry(n);
ByteArrayOutputStream bos = new ByteArrayOutputStream();
TarArchiveOutputStream tos = new TarArchiveOutputStream(bos, "ASCII");
tos.setLongFileMode(mode);
tos.putArchiveEntry(t);
tos.closeArchiveEntry();
tos.close();
byte[] data = bos.toByteArray();
TarArchiveInputStream tin =
new TarArchiveInputStream(new ByteArrayInputStream(data));
TarArchiveEntry e = tin.getNextTarEntry();
assertEquals(n, e.getName());
assertTrue(e.isDirectory());
tin.close();
}
/**
* @see https://issues.apache.org/jira/browse/COMPRESS-203
*/
public void testWriteNonAsciiDirectoryNamePosixMode() throws Exception {
String n = "f\u00f6\u00f6/";
TarArchiveEntry t = new TarArchiveEntry(n);
ByteArrayOutputStream bos = new ByteArrayOutputStream();
TarArchiveOutputStream tos = new TarArchiveOutputStream(bos);
tos.setAddPaxHeadersForNonAsciiNames(true);
tos.putArchiveEntry(t);
tos.closeArchiveEntry();
tos.close();
byte[] data = bos.toByteArray();
TarArchiveInputStream tin =
new TarArchiveInputStream(new ByteArrayInputStream(data));
TarArchiveEntry e = tin.getNextTarEntry();
assertEquals(n, e.getName());
assertTrue(e.isDirectory());
tin.close();
}
} |
|
public void testSnakeCaseWithOneArg() throws Exception
{
final String MSG = "1st";
OneProperty actual = MAPPER.readValue(
"{\"param_name0\":\""+MSG+"\"}",
OneProperty.class);
assertEquals("CTOR:"+MSG, actual.paramName0);
} | com.fasterxml.jackson.databind.deser.creators.CreatorWithNamingStrategyTest::testSnakeCaseWithOneArg | src/test/java/com/fasterxml/jackson/databind/deser/creators/CreatorWithNamingStrategyTest.java | 52 | src/test/java/com/fasterxml/jackson/databind/deser/creators/CreatorWithNamingStrategyTest.java | testSnakeCaseWithOneArg | package com.fasterxml.jackson.databind.deser.creators;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.databind.*;
import com.fasterxml.jackson.databind.introspect.AnnotatedMember;
import com.fasterxml.jackson.databind.introspect.AnnotatedParameter;
import com.fasterxml.jackson.databind.introspect.JacksonAnnotationIntrospector;
public class CreatorWithNamingStrategyTest extends BaseMapTest
{
@SuppressWarnings("serial")
static class MyParamIntrospector extends JacksonAnnotationIntrospector
{
@Override
public String findImplicitPropertyName(AnnotatedMember param) {
if (param instanceof AnnotatedParameter) {
AnnotatedParameter ap = (AnnotatedParameter) param;
return "paramName"+ap.getIndex();
}
return super.findImplicitPropertyName(param);
}
}
// [databind#2051]
static class OneProperty {
public String paramName0;
@JsonCreator
public OneProperty(String bogus) {
paramName0 = "CTOR:"+bogus;
}
}
/*
/**********************************************************
/* Test methods
/**********************************************************
*/
private final ObjectMapper MAPPER = newObjectMapper()
.setAnnotationIntrospector(new MyParamIntrospector())
.setPropertyNamingStrategy(PropertyNamingStrategy.SNAKE_CASE)
;
// [databind#2051]
public void testSnakeCaseWithOneArg() throws Exception
{
final String MSG = "1st";
OneProperty actual = MAPPER.readValue(
"{\"param_name0\":\""+MSG+"\"}",
OneProperty.class);
assertEquals("CTOR:"+MSG, actual.paramName0);
}
} | // You are a professional Java test case writer, please create a test case named `testSnakeCaseWithOneArg` for the issue `JacksonDatabind-2051`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonDatabind-2051
//
// ## Issue-Title:
// Implicit constructor property names are not renamed properly with PropertyNamingStrategy
//
// ## Issue-Description:
// (note: spin-off from [FasterXML/jackson-modules-java8#67](https://github.com/FasterXML/jackson-modules-java8/issues/67))
//
//
// Looks like something with linking of creator properties (constructor arguments for annotated/discovered constructor) to "regular" properties does not work when using `PropertyNamingStrategy`. Apparently this was working better until 2.9.1, but broke with 2.9.2.
//
//
//
//
public void testSnakeCaseWithOneArg() throws Exception {
| 52 | // [databind#2051] | 96 | 45 | src/test/java/com/fasterxml/jackson/databind/deser/creators/CreatorWithNamingStrategyTest.java | src/test/java | ```markdown
## Issue-ID: JacksonDatabind-2051
## Issue-Title:
Implicit constructor property names are not renamed properly with PropertyNamingStrategy
## Issue-Description:
(note: spin-off from [FasterXML/jackson-modules-java8#67](https://github.com/FasterXML/jackson-modules-java8/issues/67))
Looks like something with linking of creator properties (constructor arguments for annotated/discovered constructor) to "regular" properties does not work when using `PropertyNamingStrategy`. Apparently this was working better until 2.9.1, but broke with 2.9.2.
```
You are a professional Java test case writer, please create a test case named `testSnakeCaseWithOneArg` for the issue `JacksonDatabind-2051`, utilizing the provided issue report information and the following function signature.
```java
public void testSnakeCaseWithOneArg() throws Exception {
```
| 45 | [
"com.fasterxml.jackson.databind.deser.BasicDeserializerFactory"
] | 8c9a3f7e0e5f5ce3a27fc23e620c4fa45df447a558333831377125f1142058be | public void testSnakeCaseWithOneArg() throws Exception
| // You are a professional Java test case writer, please create a test case named `testSnakeCaseWithOneArg` for the issue `JacksonDatabind-2051`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonDatabind-2051
//
// ## Issue-Title:
// Implicit constructor property names are not renamed properly with PropertyNamingStrategy
//
// ## Issue-Description:
// (note: spin-off from [FasterXML/jackson-modules-java8#67](https://github.com/FasterXML/jackson-modules-java8/issues/67))
//
//
// Looks like something with linking of creator properties (constructor arguments for annotated/discovered constructor) to "regular" properties does not work when using `PropertyNamingStrategy`. Apparently this was working better until 2.9.1, but broke with 2.9.2.
//
//
//
//
| JacksonDatabind | package com.fasterxml.jackson.databind.deser.creators;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.databind.*;
import com.fasterxml.jackson.databind.introspect.AnnotatedMember;
import com.fasterxml.jackson.databind.introspect.AnnotatedParameter;
import com.fasterxml.jackson.databind.introspect.JacksonAnnotationIntrospector;
public class CreatorWithNamingStrategyTest extends BaseMapTest
{
@SuppressWarnings("serial")
static class MyParamIntrospector extends JacksonAnnotationIntrospector
{
@Override
public String findImplicitPropertyName(AnnotatedMember param) {
if (param instanceof AnnotatedParameter) {
AnnotatedParameter ap = (AnnotatedParameter) param;
return "paramName"+ap.getIndex();
}
return super.findImplicitPropertyName(param);
}
}
// [databind#2051]
static class OneProperty {
public String paramName0;
@JsonCreator
public OneProperty(String bogus) {
paramName0 = "CTOR:"+bogus;
}
}
/*
/**********************************************************
/* Test methods
/**********************************************************
*/
private final ObjectMapper MAPPER = newObjectMapper()
.setAnnotationIntrospector(new MyParamIntrospector())
.setPropertyNamingStrategy(PropertyNamingStrategy.SNAKE_CASE)
;
// [databind#2051]
public void testSnakeCaseWithOneArg() throws Exception
{
final String MSG = "1st";
OneProperty actual = MAPPER.readValue(
"{\"param_name0\":\""+MSG+"\"}",
OneProperty.class);
assertEquals("CTOR:"+MSG, actual.paramName0);
}
} |
|
@Test public void nextIndexOfUnmatched() {
CharacterReader r = new CharacterReader("<[[one]]");
assertEquals(-1, r.nextIndexOf("]]>"));
} | org.jsoup.parser.CharacterReaderTest::nextIndexOfUnmatched | src/test/java/org/jsoup/parser/CharacterReaderTest.java | 100 | src/test/java/org/jsoup/parser/CharacterReaderTest.java | nextIndexOfUnmatched | package org.jsoup.parser;
import org.junit.Test;
import static org.junit.Assert.*;
/**
* Test suite for character reader.
*
* @author Jonathan Hedley, [email protected]
*/
public class CharacterReaderTest {
@Test public void consume() {
CharacterReader r = new CharacterReader("one");
assertEquals(0, r.pos());
assertEquals('o', r.current());
assertEquals('o', r.consume());
assertEquals(1, r.pos());
assertEquals('n', r.current());
assertEquals(1, r.pos());
assertEquals('n', r.consume());
assertEquals('e', r.consume());
assertTrue(r.isEmpty());
assertEquals(CharacterReader.EOF, r.consume());
assertTrue(r.isEmpty());
assertEquals(CharacterReader.EOF, r.consume());
}
@Test public void unconsume() {
CharacterReader r = new CharacterReader("one");
assertEquals('o', r.consume());
assertEquals('n', r.current());
r.unconsume();
assertEquals('o', r.current());
assertEquals('o', r.consume());
assertEquals('n', r.consume());
assertEquals('e', r.consume());
assertTrue(r.isEmpty());
r.unconsume();
assertFalse(r.isEmpty());
assertEquals('e', r.current());
assertEquals('e', r.consume());
assertTrue(r.isEmpty());
assertEquals(CharacterReader.EOF, r.consume());
r.unconsume();
assertTrue(r.isEmpty());
assertEquals(CharacterReader.EOF, r.current());
}
@Test public void mark() {
CharacterReader r = new CharacterReader("one");
r.consume();
r.mark();
assertEquals('n', r.consume());
assertEquals('e', r.consume());
assertTrue(r.isEmpty());
r.rewindToMark();
assertEquals('n', r.consume());
}
@Test public void consumeToEnd() {
String in = "one two three";
CharacterReader r = new CharacterReader(in);
String toEnd = r.consumeToEnd();
assertEquals(in, toEnd);
assertTrue(r.isEmpty());
}
@Test public void nextIndexOfChar() {
String in = "blah blah";
CharacterReader r = new CharacterReader(in);
assertEquals(-1, r.nextIndexOf('x'));
assertEquals(3, r.nextIndexOf('h'));
String pull = r.consumeTo('h');
assertEquals("bla", pull);
r.consume();
assertEquals(2, r.nextIndexOf('l'));
assertEquals(" blah", r.consumeToEnd());
assertEquals(-1, r.nextIndexOf('x'));
}
@Test public void nextIndexOfString() {
String in = "One Two something Two Three Four";
CharacterReader r = new CharacterReader(in);
assertEquals(-1, r.nextIndexOf("Foo"));
assertEquals(4, r.nextIndexOf("Two"));
assertEquals("One Two ", r.consumeTo("something"));
assertEquals(10, r.nextIndexOf("Two"));
assertEquals("something Two Three Four", r.consumeToEnd());
assertEquals(-1, r.nextIndexOf("Two"));
}
@Test public void nextIndexOfUnmatched() {
CharacterReader r = new CharacterReader("<[[one]]");
assertEquals(-1, r.nextIndexOf("]]>"));
}
@Test public void consumeToChar() {
CharacterReader r = new CharacterReader("One Two Three");
assertEquals("One ", r.consumeTo('T'));
assertEquals("", r.consumeTo('T')); // on Two
assertEquals('T', r.consume());
assertEquals("wo ", r.consumeTo('T'));
assertEquals('T', r.consume());
assertEquals("hree", r.consumeTo('T')); // consume to end
}
@Test public void consumeToString() {
CharacterReader r = new CharacterReader("One Two Two Four");
assertEquals("One ", r.consumeTo("Two"));
assertEquals('T', r.consume());
assertEquals("wo ", r.consumeTo("Two"));
assertEquals('T', r.consume());
assertEquals("wo Four", r.consumeTo("Qux"));
}
@Test public void advance() {
CharacterReader r = new CharacterReader("One Two Three");
assertEquals('O', r.consume());
r.advance();
assertEquals('e', r.consume());
}
@Test public void consumeToAny() {
CharacterReader r = new CharacterReader("One &bar; qux");
assertEquals("One ", r.consumeToAny('&', ';'));
assertTrue(r.matches('&'));
assertTrue(r.matches("&bar;"));
assertEquals('&', r.consume());
assertEquals("bar", r.consumeToAny('&', ';'));
assertEquals(';', r.consume());
assertEquals(" qux", r.consumeToAny('&', ';'));
}
@Test public void consumeLetterSequence() {
CharacterReader r = new CharacterReader("One &bar; qux");
assertEquals("One", r.consumeLetterSequence());
assertEquals(" &", r.consumeTo("bar;"));
assertEquals("bar", r.consumeLetterSequence());
assertEquals("; qux", r.consumeToEnd());
}
@Test public void consumeLetterThenDigitSequence() {
CharacterReader r = new CharacterReader("One12 Two &bar; qux");
assertEquals("One12", r.consumeLetterThenDigitSequence());
assertEquals(' ', r.consume());
assertEquals("Two", r.consumeLetterThenDigitSequence());
assertEquals(" &bar; qux", r.consumeToEnd());
}
@Test public void matches() {
CharacterReader r = new CharacterReader("One Two Three");
assertTrue(r.matches('O'));
assertTrue(r.matches("One Two Three"));
assertTrue(r.matches("One"));
assertFalse(r.matches("one"));
assertEquals('O', r.consume());
assertFalse(r.matches("One"));
assertTrue(r.matches("ne Two Three"));
assertFalse(r.matches("ne Two Three Four"));
assertEquals("ne Two Three", r.consumeToEnd());
assertFalse(r.matches("ne"));
}
@Test
public void matchesIgnoreCase() {
CharacterReader r = new CharacterReader("One Two Three");
assertTrue(r.matchesIgnoreCase("O"));
assertTrue(r.matchesIgnoreCase("o"));
assertTrue(r.matches('O'));
assertFalse(r.matches('o'));
assertTrue(r.matchesIgnoreCase("One Two Three"));
assertTrue(r.matchesIgnoreCase("ONE two THREE"));
assertTrue(r.matchesIgnoreCase("One"));
assertTrue(r.matchesIgnoreCase("one"));
assertEquals('O', r.consume());
assertFalse(r.matchesIgnoreCase("One"));
assertTrue(r.matchesIgnoreCase("NE Two Three"));
assertFalse(r.matchesIgnoreCase("ne Two Three Four"));
assertEquals("ne Two Three", r.consumeToEnd());
assertFalse(r.matchesIgnoreCase("ne"));
}
@Test public void containsIgnoreCase() {
CharacterReader r = new CharacterReader("One TWO three");
assertTrue(r.containsIgnoreCase("two"));
assertTrue(r.containsIgnoreCase("three"));
// weird one: does not find one, because it scans for consistent case only
assertFalse(r.containsIgnoreCase("one"));
}
@Test public void matchesAny() {
char[] scan = {' ', '\n', '\t'};
CharacterReader r = new CharacterReader("One\nTwo\tThree");
assertFalse(r.matchesAny(scan));
assertEquals("One", r.consumeToAny(scan));
assertTrue(r.matchesAny(scan));
assertEquals('\n', r.consume());
assertFalse(r.matchesAny(scan));
}
} | // You are a professional Java test case writer, please create a test case named `nextIndexOfUnmatched` for the issue `Jsoup-349`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Jsoup-349
//
// ## Issue-Title:
// Parser error on commented CDATA
//
// ## Issue-Description:
// Jsoup gives the following error when trying to parse this HTML: <https://gist.github.com/felipehummel/6122799>
//
//
//
// ```
// java.lang.ArrayIndexOutOfBoundsException: 8666
// at org.jsoup.parser.CharacterReader.nextIndexOf(CharacterReader.java:92)
// at org.jsoup.parser.CharacterReader.consumeTo(CharacterReader.java:112)
// at org.jsoup.parser.TokeniserState$67.read(TokeniserState.java:1789)
// at org.jsoup.parser.Tokeniser.read(Tokeniser.java:42)
// at org.jsoup.parser.TreeBuilder.runParser(TreeBuilder.java:47)
// at org.jsoup.parser.TreeBuilder.parse(TreeBuilder.java:41)
// at org.jsoup.parser.HtmlTreeBuilder.parse(HtmlTreeBuilder.java:37)
// at org.jsoup.parser.Parser.parse(Parser.java:90)
// at org.jsoup.Jsoup.parse(Jsoup.java:58)
// ...
//
// ```
//
// The HTML is from a entry in a RSS feed. If I remove the line:
//
//
//
// ```
// // ]]
//
// ```
//
// or just the
//
// ]]
//
//
// Then it parses the HTML nicely.
//
//
// Does this syntax error should really throw an exception or it should be silently ignored?
//
//
//
//
@Test public void nextIndexOfUnmatched() {
| 100 | 34 | 97 | src/test/java/org/jsoup/parser/CharacterReaderTest.java | src/test/java | ```markdown
## Issue-ID: Jsoup-349
## Issue-Title:
Parser error on commented CDATA
## Issue-Description:
Jsoup gives the following error when trying to parse this HTML: <https://gist.github.com/felipehummel/6122799>
```
java.lang.ArrayIndexOutOfBoundsException: 8666
at org.jsoup.parser.CharacterReader.nextIndexOf(CharacterReader.java:92)
at org.jsoup.parser.CharacterReader.consumeTo(CharacterReader.java:112)
at org.jsoup.parser.TokeniserState$67.read(TokeniserState.java:1789)
at org.jsoup.parser.Tokeniser.read(Tokeniser.java:42)
at org.jsoup.parser.TreeBuilder.runParser(TreeBuilder.java:47)
at org.jsoup.parser.TreeBuilder.parse(TreeBuilder.java:41)
at org.jsoup.parser.HtmlTreeBuilder.parse(HtmlTreeBuilder.java:37)
at org.jsoup.parser.Parser.parse(Parser.java:90)
at org.jsoup.Jsoup.parse(Jsoup.java:58)
...
```
The HTML is from a entry in a RSS feed. If I remove the line:
```
// ]]
```
or just the
]]
Then it parses the HTML nicely.
Does this syntax error should really throw an exception or it should be silently ignored?
```
You are a professional Java test case writer, please create a test case named `nextIndexOfUnmatched` for the issue `Jsoup-349`, utilizing the provided issue report information and the following function signature.
```java
@Test public void nextIndexOfUnmatched() {
```
| 97 | [
"org.jsoup.parser.CharacterReader"
] | 8cc4665f65c3aae9005ae6623b38a5d43602f9c41fe2366624b2d1cc200e5ee0 | @Test public void nextIndexOfUnmatched() | // You are a professional Java test case writer, please create a test case named `nextIndexOfUnmatched` for the issue `Jsoup-349`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Jsoup-349
//
// ## Issue-Title:
// Parser error on commented CDATA
//
// ## Issue-Description:
// Jsoup gives the following error when trying to parse this HTML: <https://gist.github.com/felipehummel/6122799>
//
//
//
// ```
// java.lang.ArrayIndexOutOfBoundsException: 8666
// at org.jsoup.parser.CharacterReader.nextIndexOf(CharacterReader.java:92)
// at org.jsoup.parser.CharacterReader.consumeTo(CharacterReader.java:112)
// at org.jsoup.parser.TokeniserState$67.read(TokeniserState.java:1789)
// at org.jsoup.parser.Tokeniser.read(Tokeniser.java:42)
// at org.jsoup.parser.TreeBuilder.runParser(TreeBuilder.java:47)
// at org.jsoup.parser.TreeBuilder.parse(TreeBuilder.java:41)
// at org.jsoup.parser.HtmlTreeBuilder.parse(HtmlTreeBuilder.java:37)
// at org.jsoup.parser.Parser.parse(Parser.java:90)
// at org.jsoup.Jsoup.parse(Jsoup.java:58)
// ...
//
// ```
//
// The HTML is from a entry in a RSS feed. If I remove the line:
//
//
//
// ```
// // ]]
//
// ```
//
// or just the
//
// ]]
//
//
// Then it parses the HTML nicely.
//
//
// Does this syntax error should really throw an exception or it should be silently ignored?
//
//
//
//
| Jsoup | package org.jsoup.parser;
import org.junit.Test;
import static org.junit.Assert.*;
/**
* Test suite for character reader.
*
* @author Jonathan Hedley, [email protected]
*/
public class CharacterReaderTest {
@Test public void consume() {
CharacterReader r = new CharacterReader("one");
assertEquals(0, r.pos());
assertEquals('o', r.current());
assertEquals('o', r.consume());
assertEquals(1, r.pos());
assertEquals('n', r.current());
assertEquals(1, r.pos());
assertEquals('n', r.consume());
assertEquals('e', r.consume());
assertTrue(r.isEmpty());
assertEquals(CharacterReader.EOF, r.consume());
assertTrue(r.isEmpty());
assertEquals(CharacterReader.EOF, r.consume());
}
@Test public void unconsume() {
CharacterReader r = new CharacterReader("one");
assertEquals('o', r.consume());
assertEquals('n', r.current());
r.unconsume();
assertEquals('o', r.current());
assertEquals('o', r.consume());
assertEquals('n', r.consume());
assertEquals('e', r.consume());
assertTrue(r.isEmpty());
r.unconsume();
assertFalse(r.isEmpty());
assertEquals('e', r.current());
assertEquals('e', r.consume());
assertTrue(r.isEmpty());
assertEquals(CharacterReader.EOF, r.consume());
r.unconsume();
assertTrue(r.isEmpty());
assertEquals(CharacterReader.EOF, r.current());
}
@Test public void mark() {
CharacterReader r = new CharacterReader("one");
r.consume();
r.mark();
assertEquals('n', r.consume());
assertEquals('e', r.consume());
assertTrue(r.isEmpty());
r.rewindToMark();
assertEquals('n', r.consume());
}
@Test public void consumeToEnd() {
String in = "one two three";
CharacterReader r = new CharacterReader(in);
String toEnd = r.consumeToEnd();
assertEquals(in, toEnd);
assertTrue(r.isEmpty());
}
@Test public void nextIndexOfChar() {
String in = "blah blah";
CharacterReader r = new CharacterReader(in);
assertEquals(-1, r.nextIndexOf('x'));
assertEquals(3, r.nextIndexOf('h'));
String pull = r.consumeTo('h');
assertEquals("bla", pull);
r.consume();
assertEquals(2, r.nextIndexOf('l'));
assertEquals(" blah", r.consumeToEnd());
assertEquals(-1, r.nextIndexOf('x'));
}
@Test public void nextIndexOfString() {
String in = "One Two something Two Three Four";
CharacterReader r = new CharacterReader(in);
assertEquals(-1, r.nextIndexOf("Foo"));
assertEquals(4, r.nextIndexOf("Two"));
assertEquals("One Two ", r.consumeTo("something"));
assertEquals(10, r.nextIndexOf("Two"));
assertEquals("something Two Three Four", r.consumeToEnd());
assertEquals(-1, r.nextIndexOf("Two"));
}
@Test public void nextIndexOfUnmatched() {
CharacterReader r = new CharacterReader("<[[one]]");
assertEquals(-1, r.nextIndexOf("]]>"));
}
@Test public void consumeToChar() {
CharacterReader r = new CharacterReader("One Two Three");
assertEquals("One ", r.consumeTo('T'));
assertEquals("", r.consumeTo('T')); // on Two
assertEquals('T', r.consume());
assertEquals("wo ", r.consumeTo('T'));
assertEquals('T', r.consume());
assertEquals("hree", r.consumeTo('T')); // consume to end
}
@Test public void consumeToString() {
CharacterReader r = new CharacterReader("One Two Two Four");
assertEquals("One ", r.consumeTo("Two"));
assertEquals('T', r.consume());
assertEquals("wo ", r.consumeTo("Two"));
assertEquals('T', r.consume());
assertEquals("wo Four", r.consumeTo("Qux"));
}
@Test public void advance() {
CharacterReader r = new CharacterReader("One Two Three");
assertEquals('O', r.consume());
r.advance();
assertEquals('e', r.consume());
}
@Test public void consumeToAny() {
CharacterReader r = new CharacterReader("One &bar; qux");
assertEquals("One ", r.consumeToAny('&', ';'));
assertTrue(r.matches('&'));
assertTrue(r.matches("&bar;"));
assertEquals('&', r.consume());
assertEquals("bar", r.consumeToAny('&', ';'));
assertEquals(';', r.consume());
assertEquals(" qux", r.consumeToAny('&', ';'));
}
@Test public void consumeLetterSequence() {
CharacterReader r = new CharacterReader("One &bar; qux");
assertEquals("One", r.consumeLetterSequence());
assertEquals(" &", r.consumeTo("bar;"));
assertEquals("bar", r.consumeLetterSequence());
assertEquals("; qux", r.consumeToEnd());
}
@Test public void consumeLetterThenDigitSequence() {
CharacterReader r = new CharacterReader("One12 Two &bar; qux");
assertEquals("One12", r.consumeLetterThenDigitSequence());
assertEquals(' ', r.consume());
assertEquals("Two", r.consumeLetterThenDigitSequence());
assertEquals(" &bar; qux", r.consumeToEnd());
}
@Test public void matches() {
CharacterReader r = new CharacterReader("One Two Three");
assertTrue(r.matches('O'));
assertTrue(r.matches("One Two Three"));
assertTrue(r.matches("One"));
assertFalse(r.matches("one"));
assertEquals('O', r.consume());
assertFalse(r.matches("One"));
assertTrue(r.matches("ne Two Three"));
assertFalse(r.matches("ne Two Three Four"));
assertEquals("ne Two Three", r.consumeToEnd());
assertFalse(r.matches("ne"));
}
@Test
public void matchesIgnoreCase() {
CharacterReader r = new CharacterReader("One Two Three");
assertTrue(r.matchesIgnoreCase("O"));
assertTrue(r.matchesIgnoreCase("o"));
assertTrue(r.matches('O'));
assertFalse(r.matches('o'));
assertTrue(r.matchesIgnoreCase("One Two Three"));
assertTrue(r.matchesIgnoreCase("ONE two THREE"));
assertTrue(r.matchesIgnoreCase("One"));
assertTrue(r.matchesIgnoreCase("one"));
assertEquals('O', r.consume());
assertFalse(r.matchesIgnoreCase("One"));
assertTrue(r.matchesIgnoreCase("NE Two Three"));
assertFalse(r.matchesIgnoreCase("ne Two Three Four"));
assertEquals("ne Two Three", r.consumeToEnd());
assertFalse(r.matchesIgnoreCase("ne"));
}
@Test public void containsIgnoreCase() {
CharacterReader r = new CharacterReader("One TWO three");
assertTrue(r.containsIgnoreCase("two"));
assertTrue(r.containsIgnoreCase("three"));
// weird one: does not find one, because it scans for consistent case only
assertFalse(r.containsIgnoreCase("one"));
}
@Test public void matchesAny() {
char[] scan = {' ', '\n', '\t'};
CharacterReader r = new CharacterReader("One\nTwo\tThree");
assertFalse(r.matchesAny(scan));
assertEquals("One", r.consumeToAny(scan));
assertTrue(r.matchesAny(scan));
assertEquals('\n', r.consume());
assertFalse(r.matchesAny(scan));
}
} |
||
@Test
public void testNoHeaderMap() throws Exception {
final CSVParser parser = CSVParser.parse("a,b,c\n1,2,3\nx,y,z", CSVFormat.DEFAULT);
Assert.assertNull(parser.getHeaderMap());
} | org.apache.commons.csv.CSVParserTest::testNoHeaderMap | src/test/java/org/apache/commons/csv/CSVParserTest.java | 670 | src/test/java/org/apache/commons/csv/CSVParserTest.java | testNoHeaderMap | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.csv;
import static org.apache.commons.csv.Constants.CR;
import static org.apache.commons.csv.Constants.CRLF;
import static org.apache.commons.csv.Constants.LF;
import static org.junit.Assert.assertArrayEquals;
import static org.junit.Assert.assertEquals;
import static org.junit.Assert.assertFalse;
import static org.junit.Assert.assertNotNull;
import static org.junit.Assert.assertNull;
import static org.junit.Assert.assertTrue;
import static org.junit.Assert.fail;
import java.io.File;
import java.io.IOException;
import java.io.Reader;
import java.io.StringReader;
import java.io.StringWriter;
import java.net.URL;
import java.nio.charset.Charset;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.NoSuchElementException;
import org.junit.Assert;
import org.junit.Ignore;
import org.junit.Test;
/**
* CSVParserTest
*
* The test are organized in three different sections:
* The 'setter/getter' section, the lexer section and finally the parser
* section. In case a test fails, you should follow a top-down approach for
* fixing a potential bug (its likely that the parser itself fails if the lexer
* has problems...).
*
* @version $Id$
*/
public class CSVParserTest {
private static final String CSVINPUT = "a,b,c,d\n"
+ " a , b , 1 2 \n"
+ "\"foo baar\", b,\n"
// + " \"foo\n,,\n\"\",,\n\\\"\",d,e\n";
+ " \"foo\n,,\n\"\",,\n\"\"\",d,e\n"; // changed to use standard CSV escaping
private static final String[][] RESULT = {
{"a", "b", "c", "d"},
{"a", "b", "1 2"},
{"foo baar", "b", ""},
{"foo\n,,\n\",,\n\"", "d", "e"}
};
@Test
public void testGetLine() throws IOException {
final CSVParser parser = CSVParser.parse(CSVINPUT, CSVFormat.DEFAULT.withIgnoreSurroundingSpaces(true));
for (final String[] re : RESULT) {
assertArrayEquals(re, parser.nextRecord().values());
}
assertNull(parser.nextRecord());
}
@Test
public void testGetRecords() throws IOException {
final CSVParser parser = CSVParser.parse(CSVINPUT, CSVFormat.DEFAULT.withIgnoreSurroundingSpaces(true));
final List<CSVRecord> records = parser.getRecords();
assertEquals(RESULT.length, records.size());
assertTrue(records.size() > 0);
for (int i = 0; i < RESULT.length; i++) {
assertArrayEquals(RESULT[i], records.get(i).values());
}
}
@Test
public void testExcelFormat1() throws IOException {
final String code =
"value1,value2,value3,value4\r\na,b,c,d\r\n x,,,"
+ "\r\n\r\n\"\"\"hello\"\"\",\" \"\"world\"\"\",\"abc\ndef\",\r\n";
final String[][] res = {
{"value1", "value2", "value3", "value4"},
{"a", "b", "c", "d"},
{" x", "", "", ""},
{""},
{"\"hello\"", " \"world\"", "abc\ndef", ""}
};
final CSVParser parser = CSVParser.parse(code, CSVFormat.EXCEL);
final List<CSVRecord> records = parser.getRecords();
assertEquals(res.length, records.size());
assertTrue(records.size() > 0);
for (int i = 0; i < res.length; i++) {
assertArrayEquals(res[i], records.get(i).values());
}
}
@Test
public void testExcelFormat2() throws Exception {
final String code = "foo,baar\r\n\r\nhello,\r\n\r\nworld,\r\n";
final String[][] res = {
{"foo", "baar"},
{""},
{"hello", ""},
{""},
{"world", ""}
};
final CSVParser parser = CSVParser.parse(code, CSVFormat.EXCEL);
final List<CSVRecord> records = parser.getRecords();
assertEquals(res.length, records.size());
assertTrue(records.size() > 0);
for (int i = 0; i < res.length; i++) {
assertArrayEquals(res[i], records.get(i).values());
}
}
@Test
public void testEndOfFileBehaviourExcel() throws Exception {
final String[] codes = {
"hello,\r\n\r\nworld,\r\n",
"hello,\r\n\r\nworld,",
"hello,\r\n\r\nworld,\"\"\r\n",
"hello,\r\n\r\nworld,\"\"",
"hello,\r\n\r\nworld,\n",
"hello,\r\n\r\nworld,",
"hello,\r\n\r\nworld,\"\"\n",
"hello,\r\n\r\nworld,\"\""
};
final String[][] res = {
{"hello", ""},
{""}, // Excel format does not ignore empty lines
{"world", ""}
};
for (final String code : codes) {
final CSVParser parser = CSVParser.parse(code, CSVFormat.EXCEL);
final List<CSVRecord> records = parser.getRecords();
assertEquals(res.length, records.size());
assertTrue(records.size() > 0);
for (int i = 0; i < res.length; i++) {
assertArrayEquals(res[i], records.get(i).values());
}
}
}
@Test
public void testEndOfFileBehaviorCSV() throws Exception {
final String[] codes = {
"hello,\r\n\r\nworld,\r\n",
"hello,\r\n\r\nworld,",
"hello,\r\n\r\nworld,\"\"\r\n",
"hello,\r\n\r\nworld,\"\"",
"hello,\r\n\r\nworld,\n",
"hello,\r\n\r\nworld,",
"hello,\r\n\r\nworld,\"\"\n",
"hello,\r\n\r\nworld,\"\""
};
final String[][] res = {
{"hello", ""}, // CSV format ignores empty lines
{"world", ""}
};
for (final String code : codes) {
final CSVParser parser = CSVParser.parse(code, CSVFormat.DEFAULT);
final List<CSVRecord> records = parser.getRecords();
assertEquals(res.length, records.size());
assertTrue(records.size() > 0);
for (int i = 0; i < res.length; i++) {
assertArrayEquals(res[i], records.get(i).values());
}
}
}
@Test
public void testEmptyLineBehaviourExcel() throws Exception {
final String[] codes = {
"hello,\r\n\r\n\r\n",
"hello,\n\n\n",
"hello,\"\"\r\n\r\n\r\n",
"hello,\"\"\n\n\n"
};
final String[][] res = {
{"hello", ""},
{""}, // Excel format does not ignore empty lines
{""}
};
for (final String code : codes) {
final CSVParser parser = CSVParser.parse(code, CSVFormat.EXCEL);
final List<CSVRecord> records = parser.getRecords();
assertEquals(res.length, records.size());
assertTrue(records.size() > 0);
for (int i = 0; i < res.length; i++) {
assertArrayEquals(res[i], records.get(i).values());
}
}
}
@Test
public void testEmptyLineBehaviourCSV() throws Exception {
final String[] codes = {
"hello,\r\n\r\n\r\n",
"hello,\n\n\n",
"hello,\"\"\r\n\r\n\r\n",
"hello,\"\"\n\n\n"
};
final String[][] res = {
{"hello", ""} // CSV format ignores empty lines
};
for (final String code : codes) {
final CSVParser parser = CSVParser.parse(code, CSVFormat.DEFAULT);
final List<CSVRecord> records = parser.getRecords();
assertEquals(res.length, records.size());
assertTrue(records.size() > 0);
for (int i = 0; i < res.length; i++) {
assertArrayEquals(res[i], records.get(i).values());
}
}
}
@Test
public void testEmptyFile() throws Exception {
final CSVParser parser = CSVParser.parse("", CSVFormat.DEFAULT);
assertNull(parser.nextRecord());
}
@Test
public void testCSV57() throws Exception {
final CSVParser parser = CSVParser.parse("", CSVFormat.DEFAULT);
final List<CSVRecord> list = parser.getRecords();
assertNotNull(list);
assertEquals(0, list.size());
}
@Test
@Ignore
public void testBackslashEscapingOld() throws IOException {
final String code =
"one,two,three\n"
+ "on\\\"e,two\n"
+ "on\"e,two\n"
+ "one,\"tw\\\"o\"\n"
+ "one,\"t\\,wo\"\n"
+ "one,two,\"th,ree\"\n"
+ "\"a\\\\\"\n"
+ "a\\,b\n"
+ "\"a\\\\,b\"";
final String[][] res = {
{"one", "two", "three"},
{"on\\\"e", "two"},
{"on\"e", "two"},
{"one", "tw\"o"},
{"one", "t\\,wo"}, // backslash in quotes only escapes a delimiter (",")
{"one", "two", "th,ree"},
{"a\\\\"}, // backslash in quotes only escapes a delimiter (",")
{"a\\", "b"}, // a backslash must be returnd
{"a\\\\,b"} // backslash in quotes only escapes a delimiter (",")
};
final CSVParser parser = CSVParser.parse(code, CSVFormat.DEFAULT);
final List<CSVRecord> records = parser.getRecords();
assertEquals(res.length, records.size());
assertTrue(records.size() > 0);
for (int i = 0; i < res.length; i++) {
assertArrayEquals(res[i], records.get(i).values());
}
}
@Test
public void testBackslashEscaping() throws IOException {
// To avoid confusion over the need for escaping chars in java code,
// We will test with a forward slash as the escape char, and a single
// quote as the encapsulator.
final String code =
"one,two,three\n" // 0
+ "'',''\n" // 1) empty encapsulators
+ "/',/'\n" // 2) single encapsulators
+ "'/'','/''\n" // 3) single encapsulators encapsulated via escape
+ "'''',''''\n" // 4) single encapsulators encapsulated via doubling
+ "/,,/,\n" // 5) separator escaped
+ "//,//\n" // 6) escape escaped
+ "'//','//'\n" // 7) escape escaped in encapsulation
+ " 8 , \"quoted \"\" /\" // string\" \n" // don't eat spaces
+ "9, /\n \n" // escaped newline
+ "";
final String[][] res = {
{"one", "two", "three"}, // 0
{"", ""}, // 1
{"'", "'"}, // 2
{"'", "'"}, // 3
{"'", "'"}, // 4
{",", ","}, // 5
{"/", "/"}, // 6
{"/", "/"}, // 7
{" 8 ", " \"quoted \"\" /\" / string\" "},
{"9", " \n "},
};
final CSVFormat format = CSVFormat.newFormat(',').withQuoteChar('\'')
.withRecordSeparator(CRLF).withEscape('/').withIgnoreEmptyLines(true);
final CSVParser parser = CSVParser.parse(code, format);
final List<CSVRecord> records = parser.getRecords();
assertTrue(records.size() > 0);
Utils.compare("Records do not match expected result", res, records);
}
@Test
public void testBackslashEscaping2() throws IOException {
// To avoid confusion over the need for escaping chars in java code,
// We will test with a forward slash as the escape char, and a single
// quote as the encapsulator.
final String code = ""
+ " , , \n" // 1)
+ " \t , , \n" // 2)
+ " // , /, , /,\n" // 3)
+ "";
final String[][] res = {
{" ", " ", " "}, // 1
{" \t ", " ", " "}, // 2
{" / ", " , ", " ,"}, // 3
};
final CSVFormat format = CSVFormat.newFormat(',')
.withRecordSeparator(CRLF).withEscape('/').withIgnoreEmptyLines(true);
final CSVParser parser = CSVParser.parse(code, format);
final List<CSVRecord> records = parser.getRecords();
assertTrue(records.size() > 0);
Utils.compare("", res, records);
}
@Test
public void testDefaultFormat() throws IOException {
final String code = ""
+ "a,b#\n" // 1)
+ "\"\n\",\" \",#\n" // 2)
+ "#,\"\"\n" // 3)
+ "# Final comment\n"// 4)
;
final String[][] res = {
{"a", "b#"},
{"\n", " ", "#"},
{"#", ""},
{"# Final comment"}
};
CSVFormat format = CSVFormat.DEFAULT;
assertFalse(format.isCommentingEnabled());
CSVParser parser = CSVParser.parse(code, format);
List<CSVRecord> records = parser.getRecords();
assertTrue(records.size() > 0);
Utils.compare("Failed to parse without comments", res, records);
final String[][] res_comments = {
{"a", "b#"},
{"\n", " ", "#"},
};
format = CSVFormat.DEFAULT.withCommentStart('#');
parser = CSVParser.parse(code, format);
records = parser.getRecords();
Utils.compare("Failed to parse with comments", res_comments, records);
}
@Test
public void testCarriageReturnLineFeedEndings() throws IOException {
final String code = "foo\r\nbaar,\r\nhello,world\r\n,kanu";
final CSVParser parser = CSVParser.parse(code, CSVFormat.DEFAULT);
final List<CSVRecord> records = parser.getRecords();
assertEquals(4, records.size());
}
@Test(expected = NoSuchElementException.class)
public void testClose() throws Exception {
final Reader in = new StringReader("# comment\na,b,c\n1,2,3\nx,y,z");
final CSVParser parser = CSVFormat.DEFAULT.withCommentStart('#').withHeader().parse(in);
final Iterator<CSVRecord> records = parser.iterator();
assertTrue(records.hasNext());
parser.close();
assertFalse(records.hasNext());
records.next();
}
@Test
public void testCarriageReturnEndings() throws IOException {
final String code = "foo\rbaar,\rhello,world\r,kanu";
final CSVParser parser = CSVParser.parse(code, CSVFormat.DEFAULT);
final List<CSVRecord> records = parser.getRecords();
assertEquals(4, records.size());
}
@Test
public void testLineFeedEndings() throws IOException {
final String code = "foo\nbaar,\nhello,world\n,kanu";
final CSVParser parser = CSVParser.parse(code, CSVFormat.DEFAULT);
final List<CSVRecord> records = parser.getRecords();
assertEquals(4, records.size());
}
@Test
public void testIgnoreEmptyLines() throws IOException {
final String code = "\nfoo,baar\n\r\n,\n\n,world\r\n\n";
//String code = "world\r\n\n";
//String code = "foo;baar\r\n\r\nhello;\r\n\r\nworld;\r\n";
final CSVParser parser = CSVParser.parse(code, CSVFormat.DEFAULT);
final List<CSVRecord> records = parser.getRecords();
assertEquals(3, records.size());
}
@Test
public void testForEach() throws Exception {
final List<CSVRecord> records = new ArrayList<CSVRecord>();
final Reader in = new StringReader("a,b,c\n1,2,3\nx,y,z");
for (final CSVRecord record : CSVFormat.DEFAULT.parse(in)) {
records.add(record);
}
assertEquals(3, records.size());
assertArrayEquals(new String[]{"a", "b", "c"}, records.get(0).values());
assertArrayEquals(new String[]{"1", "2", "3"}, records.get(1).values());
assertArrayEquals(new String[]{"x", "y", "z"}, records.get(2).values());
}
@Test
public void testRoundtrip() throws Exception {
final StringWriter out = new StringWriter();
final CSVPrinter printer = new CSVPrinter(out, CSVFormat.DEFAULT);
final String input = "a,b,c\r\n1,2,3\r\nx,y,z\r\n";
for (final CSVRecord record : CSVParser.parse(input, CSVFormat.DEFAULT)) {
printer.printRecord(record);
}
assertEquals(input, out.toString());
printer.close();
}
@Test
public void testIterator() throws Exception {
final Reader in = new StringReader("a,b,c\n1,2,3\nx,y,z");
final Iterator<CSVRecord> iterator = CSVFormat.DEFAULT.parse(in).iterator();
assertTrue(iterator.hasNext());
try {
iterator.remove();
fail("expected UnsupportedOperationException");
} catch (final UnsupportedOperationException expected) {
// expected
}
assertArrayEquals(new String[]{"a", "b", "c"}, iterator.next().values());
assertArrayEquals(new String[]{"1", "2", "3"}, iterator.next().values());
assertTrue(iterator.hasNext());
assertTrue(iterator.hasNext());
assertTrue(iterator.hasNext());
assertArrayEquals(new String[]{"x", "y", "z"}, iterator.next().values());
assertFalse(iterator.hasNext());
try {
iterator.next();
fail("NoSuchElementException expected");
} catch (final NoSuchElementException e) {
// expected
}
}
@Test // TODO this may lead to strange behavior, throw an exception if iterator() has already been called?
public void testMultipleIterators() throws Exception {
CSVParser parser = CSVParser.parse("a,b,c" + CR + "d,e,f", CSVFormat.DEFAULT);
Iterator<CSVRecord> itr1 = parser.iterator();
Iterator<CSVRecord> itr2 = parser.iterator();
CSVRecord first = itr1.next();
assertEquals("a", first.get(0));
assertEquals("b", first.get(1));
assertEquals("c", first.get(2));
CSVRecord second = itr2.next();
assertEquals("d", second.get(0));
assertEquals("e", second.get(1));
assertEquals("f", second.get(2));
}
@Test
public void testHeader() throws Exception {
final Reader in = new StringReader("a,b,c\n1,2,3\nx,y,z");
final Iterator<CSVRecord> records = CSVFormat.DEFAULT.withHeader().parse(in).iterator();
for (int i = 0; i < 2; i++) {
assertTrue(records.hasNext());
final CSVRecord record = records.next();
assertEquals(record.get(0), record.get("a"));
assertEquals(record.get(1), record.get("b"));
assertEquals(record.get(2), record.get("c"));
}
assertFalse(records.hasNext());
}
@Test
public void testSkipSetHeader() throws Exception {
final Reader in = new StringReader("a,b,c\n1,2,3\nx,y,z");
final Iterator<CSVRecord> records = CSVFormat.DEFAULT.withHeader("a", "b", "c").withSkipHeaderRecord(true)
.parse(in).iterator();
final CSVRecord record = records.next();
assertEquals("1", record.get("a"));
assertEquals("2", record.get("b"));
assertEquals("3", record.get("c"));
}
@Test
public void testSkipAutoHeader() throws Exception {
final Reader in = new StringReader("a,b,c\n1,2,3\nx,y,z");
final Iterator<CSVRecord> records = CSVFormat.DEFAULT.withHeader().parse(in).iterator();
final CSVRecord record = records.next();
assertEquals("1", record.get("a"));
assertEquals("2", record.get("b"));
assertEquals("3", record.get("c"));
}
@Test
public void testHeaderComment() throws Exception {
final Reader in = new StringReader("# comment\na,b,c\n1,2,3\nx,y,z");
final Iterator<CSVRecord> records = CSVFormat.DEFAULT.withCommentStart('#').withHeader().parse(in).iterator();
for (int i = 0; i < 2; i++) {
assertTrue(records.hasNext());
final CSVRecord record = records.next();
assertEquals(record.get(0), record.get("a"));
assertEquals(record.get(1), record.get("b"));
assertEquals(record.get(2), record.get("c"));
}
assertFalse(records.hasNext());
}
@Test
public void testProvidedHeader() throws Exception {
final Reader in = new StringReader("a,b,c\n1,2,3\nx,y,z");
final Iterator<CSVRecord> records = CSVFormat.DEFAULT.withHeader("A", "B", "C").parse(in).iterator();
for (int i = 0; i < 3; i++) {
assertTrue(records.hasNext());
final CSVRecord record = records.next();
assertTrue(record.isMapped("A"));
assertTrue(record.isMapped("B"));
assertTrue(record.isMapped("C"));
assertFalse(record.isMapped("NOT MAPPED"));
assertEquals(record.get(0), record.get("A"));
assertEquals(record.get(1), record.get("B"));
assertEquals(record.get(2), record.get("C"));
}
assertFalse(records.hasNext());
}
@Test
public void testProvidedHeaderAuto() throws Exception {
final Reader in = new StringReader("a,b,c\n1,2,3\nx,y,z");
final Iterator<CSVRecord> records = CSVFormat.DEFAULT.withHeader().parse(in).iterator();
for (int i = 0; i < 2; i++) {
assertTrue(records.hasNext());
final CSVRecord record = records.next();
assertTrue(record.isMapped("a"));
assertTrue(record.isMapped("b"));
assertTrue(record.isMapped("c"));
assertFalse(record.isMapped("NOT MAPPED"));
assertEquals(record.get(0), record.get("a"));
assertEquals(record.get(1), record.get("b"));
assertEquals(record.get(2), record.get("c"));
}
assertFalse(records.hasNext());
}
@Test
public void testMappedButNotSetAsOutlook2007ContactExport() throws Exception {
final Reader in = new StringReader("a,b,c\n1,2\nx,y,z");
final Iterator<CSVRecord> records = CSVFormat.DEFAULT.withHeader("A", "B", "C").withSkipHeaderRecord(true)
.parse(in).iterator();
CSVRecord record;
// 1st record
record = records.next();
assertTrue(record.isMapped("A"));
assertTrue(record.isMapped("B"));
assertTrue(record.isMapped("C"));
assertTrue(record.isSet("A"));
assertTrue(record.isSet("B"));
assertFalse(record.isSet("C"));
assertEquals("1", record.get("A"));
assertEquals("2", record.get("B"));
assertFalse(record.isConsistent());
// 2nd record
record = records.next();
assertTrue(record.isMapped("A"));
assertTrue(record.isMapped("B"));
assertTrue(record.isMapped("C"));
assertTrue(record.isSet("A"));
assertTrue(record.isSet("B"));
assertTrue(record.isSet("C"));
assertEquals("x", record.get("A"));
assertEquals("y", record.get("B"));
assertEquals("z", record.get("C"));
assertTrue(record.isConsistent());
assertFalse(records.hasNext());
}
@Test
public void testGetHeaderMap() throws Exception {
final CSVParser parser = CSVParser.parse("a,b,c\n1,2,3\nx,y,z", CSVFormat.DEFAULT.withHeader("A", "B", "C"));
final Map<String, Integer> headerMap = parser.getHeaderMap();
final Iterator<String> columnNames = headerMap.keySet().iterator();
// Headers are iterated in column order.
Assert.assertEquals("A", columnNames.next());
Assert.assertEquals("B", columnNames.next());
Assert.assertEquals("C", columnNames.next());
final Iterator<CSVRecord> records = parser.iterator();
// Parse to make sure getHeaderMap did not have a side-effect.
for (int i = 0; i < 3; i++) {
assertTrue(records.hasNext());
final CSVRecord record = records.next();
assertEquals(record.get(0), record.get("A"));
assertEquals(record.get(1), record.get("B"));
assertEquals(record.get(2), record.get("C"));
}
assertFalse(records.hasNext());
}
@Test
public void testNoHeaderMap() throws Exception {
final CSVParser parser = CSVParser.parse("a,b,c\n1,2,3\nx,y,z", CSVFormat.DEFAULT);
Assert.assertNull(parser.getHeaderMap());
}
@Test
public void testGetLineNumberWithLF() throws Exception {
this.validateLineNumbers(String.valueOf(LF));
}
@Test
public void testGetLineNumberWithCRLF() throws Exception {
this.validateLineNumbers(CRLF);
}
@Test
public void testGetLineNumberWithCR() throws Exception {
this.validateLineNumbers(String.valueOf(CR));
}
@Test
public void testGetRecordNumberWithLF() throws Exception {
this.validateRecordNumbers(String.valueOf(LF));
}
@Test
public void testGetRecordWithMultiLineValues() throws Exception {
final CSVParser parser = CSVParser.parse("\"a\r\n1\",\"a\r\n2\"" + CRLF + "\"b\r\n1\",\"b\r\n2\"" + CRLF + "\"c\r\n1\",\"c\r\n2\"",
CSVFormat.DEFAULT.withRecordSeparator(CRLF));
CSVRecord record;
assertEquals(0, parser.getRecordNumber());
assertEquals(0, parser.getCurrentLineNumber());
assertNotNull(record = parser.nextRecord());
assertEquals(3, parser.getCurrentLineNumber());
assertEquals(1, record.getRecordNumber());
assertEquals(1, parser.getRecordNumber());
assertNotNull(record = parser.nextRecord());
assertEquals(6, parser.getCurrentLineNumber());
assertEquals(2, record.getRecordNumber());
assertEquals(2, parser.getRecordNumber());
assertNotNull(record = parser.nextRecord());
assertEquals(8, parser.getCurrentLineNumber());
assertEquals(3, record.getRecordNumber());
assertEquals(3, parser.getRecordNumber());
assertNull(record = parser.nextRecord());
assertEquals(8, parser.getCurrentLineNumber());
assertEquals(3, parser.getRecordNumber());
}
@Test
public void testGetRecordNumberWithCRLF() throws Exception {
this.validateRecordNumbers(CRLF);
}
@Test
public void testGetRecordNumberWithCR() throws Exception {
this.validateRecordNumbers(String.valueOf(CR));
}
@Test(expected = IllegalArgumentException.class)
public void testInvalidFormat() throws Exception {
final CSVFormat invalidFormat = CSVFormat.DEFAULT.withDelimiter(CR);
new CSVParser(null, invalidFormat).close();
}
@Test(expected = IllegalArgumentException.class)
public void testParseNullFileFormat() throws Exception {
CSVParser.parse((File) null, CSVFormat.DEFAULT);
}
@Test(expected = IllegalArgumentException.class)
public void testParseFileNullFormat() throws Exception {
CSVParser.parse(new File(""), null);
}
@Test(expected = IllegalArgumentException.class)
public void testParseNullStringFormat() throws Exception {
CSVParser.parse((String) null, CSVFormat.DEFAULT);
}
@Test(expected = IllegalArgumentException.class)
public void testParseStringNullFormat() throws Exception {
CSVParser.parse("csv data", null);
}
@Test(expected = IllegalArgumentException.class)
public void testParseNullUrlCharsetFormat() throws Exception {
CSVParser.parse(null, Charset.defaultCharset(), CSVFormat.DEFAULT);
}
@Test(expected = IllegalArgumentException.class)
public void testParserUrlNullCharsetFormat() throws Exception {
CSVParser.parse(new URL("http://commons.apache.org"), null, CSVFormat.DEFAULT);
}
@Test(expected = IllegalArgumentException.class)
public void testParseUrlCharsetNullFormat() throws Exception {
CSVParser.parse(new URL("http://commons.apache.org"), Charset.defaultCharset(), null);
}
@Test(expected = IllegalArgumentException.class)
public void testNewCSVParserNullReaderFormat() throws Exception {
new CSVParser(null, CSVFormat.DEFAULT);
}
@Test(expected = IllegalArgumentException.class)
public void testNewCSVParserReaderNullFormat() throws Exception {
new CSVParser(new StringReader(""), null);
}
private void validateRecordNumbers(final String lineSeparator) throws IOException {
final CSVParser parser = CSVParser.parse("a" + lineSeparator + "b" + lineSeparator + "c", CSVFormat.DEFAULT.withRecordSeparator(lineSeparator));
CSVRecord record;
assertEquals(0, parser.getRecordNumber());
assertNotNull(record = parser.nextRecord());
assertEquals(1, record.getRecordNumber());
assertEquals(1, parser.getRecordNumber());
assertNotNull(record = parser.nextRecord());
assertEquals(2, record.getRecordNumber());
assertEquals(2, parser.getRecordNumber());
assertNotNull(record = parser.nextRecord());
assertEquals(3, record.getRecordNumber());
assertEquals(3, parser.getRecordNumber());
assertNull(record = parser.nextRecord());
assertEquals(3, parser.getRecordNumber());
}
private void validateLineNumbers(final String lineSeparator) throws IOException {
final CSVParser parser = CSVParser.parse("a" + lineSeparator + "b" + lineSeparator + "c", CSVFormat.DEFAULT.withRecordSeparator(lineSeparator));
assertEquals(0, parser.getCurrentLineNumber());
assertNotNull(parser.nextRecord());
assertEquals(1, parser.getCurrentLineNumber());
assertNotNull(parser.nextRecord());
assertEquals(2, parser.getCurrentLineNumber());
assertNotNull(parser.nextRecord());
// Still 2 because the last line is does not have EOL chars
assertEquals(2, parser.getCurrentLineNumber());
assertNull(parser.nextRecord());
// Still 2 because the last line is does not have EOL chars
assertEquals(2, parser.getCurrentLineNumber());
}
} | // You are a professional Java test case writer, please create a test case named `testNoHeaderMap` for the issue `Csv-CSV-100`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Csv-CSV-100
//
// ## Issue-Title:
// CSVParser: getHeaderMap throws NPE
//
// ## Issue-Description:
//
// title nearly says it all 
//
//
// Given a CSVParser parser, the following line throws an NPE:
//
//
//
//
// ```
// Map<String, Integer> header = parser.getHeaderMap();
//
// ```
//
//
// Stacktrace:
//
//
//
//
// ```
// Caused by: java.lang.NullPointerException
// at java.util.HashMap.<init>(HashMap.java:318)
// at java.util.LinkedHashMap.<init>(LinkedHashMap.java:212)
// at org.apache.commons.csv.CSVParser.getHeaderMap(CSVParser.java:288)
//
// ```
//
//
// happens if the format doesn't have a headerMap.
//
//
// to fix, check if the parser's headerMap is null before trying to create the returned map:
//
//
//
//
// ```
// public Map<String, Integer> getHeaderMap() {
// return this.headerMap != null ?
// new LinkedHashMap<String, Integer>(this.headerMap)
// : null;
// }
//
//
// ```
//
//
//
//
//
@Test
public void testNoHeaderMap() throws Exception {
| 670 | 4 | 666 | src/test/java/org/apache/commons/csv/CSVParserTest.java | src/test/java | ```markdown
## Issue-ID: Csv-CSV-100
## Issue-Title:
CSVParser: getHeaderMap throws NPE
## Issue-Description:
title nearly says it all 
Given a CSVParser parser, the following line throws an NPE:
```
Map<String, Integer> header = parser.getHeaderMap();
```
Stacktrace:
```
Caused by: java.lang.NullPointerException
at java.util.HashMap.<init>(HashMap.java:318)
at java.util.LinkedHashMap.<init>(LinkedHashMap.java:212)
at org.apache.commons.csv.CSVParser.getHeaderMap(CSVParser.java:288)
```
happens if the format doesn't have a headerMap.
to fix, check if the parser's headerMap is null before trying to create the returned map:
```
public Map<String, Integer> getHeaderMap() {
return this.headerMap != null ?
new LinkedHashMap<String, Integer>(this.headerMap)
: null;
}
```
```
You are a professional Java test case writer, please create a test case named `testNoHeaderMap` for the issue `Csv-CSV-100`, utilizing the provided issue report information and the following function signature.
```java
@Test
public void testNoHeaderMap() throws Exception {
```
| 666 | [
"org.apache.commons.csv.CSVParser"
] | 8ced1e9d4de16bc1057c225b947cb19f104b252e6eda62c13abbe21f7e2935c1 | @Test
public void testNoHeaderMap() throws Exception | // You are a professional Java test case writer, please create a test case named `testNoHeaderMap` for the issue `Csv-CSV-100`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Csv-CSV-100
//
// ## Issue-Title:
// CSVParser: getHeaderMap throws NPE
//
// ## Issue-Description:
//
// title nearly says it all 
//
//
// Given a CSVParser parser, the following line throws an NPE:
//
//
//
//
// ```
// Map<String, Integer> header = parser.getHeaderMap();
//
// ```
//
//
// Stacktrace:
//
//
//
//
// ```
// Caused by: java.lang.NullPointerException
// at java.util.HashMap.<init>(HashMap.java:318)
// at java.util.LinkedHashMap.<init>(LinkedHashMap.java:212)
// at org.apache.commons.csv.CSVParser.getHeaderMap(CSVParser.java:288)
//
// ```
//
//
// happens if the format doesn't have a headerMap.
//
//
// to fix, check if the parser's headerMap is null before trying to create the returned map:
//
//
//
//
// ```
// public Map<String, Integer> getHeaderMap() {
// return this.headerMap != null ?
// new LinkedHashMap<String, Integer>(this.headerMap)
// : null;
// }
//
//
// ```
//
//
//
//
//
| Csv | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.csv;
import static org.apache.commons.csv.Constants.CR;
import static org.apache.commons.csv.Constants.CRLF;
import static org.apache.commons.csv.Constants.LF;
import static org.junit.Assert.assertArrayEquals;
import static org.junit.Assert.assertEquals;
import static org.junit.Assert.assertFalse;
import static org.junit.Assert.assertNotNull;
import static org.junit.Assert.assertNull;
import static org.junit.Assert.assertTrue;
import static org.junit.Assert.fail;
import java.io.File;
import java.io.IOException;
import java.io.Reader;
import java.io.StringReader;
import java.io.StringWriter;
import java.net.URL;
import java.nio.charset.Charset;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.NoSuchElementException;
import org.junit.Assert;
import org.junit.Ignore;
import org.junit.Test;
/**
* CSVParserTest
*
* The test are organized in three different sections:
* The 'setter/getter' section, the lexer section and finally the parser
* section. In case a test fails, you should follow a top-down approach for
* fixing a potential bug (its likely that the parser itself fails if the lexer
* has problems...).
*
* @version $Id$
*/
public class CSVParserTest {
private static final String CSVINPUT = "a,b,c,d\n"
+ " a , b , 1 2 \n"
+ "\"foo baar\", b,\n"
// + " \"foo\n,,\n\"\",,\n\\\"\",d,e\n";
+ " \"foo\n,,\n\"\",,\n\"\"\",d,e\n"; // changed to use standard CSV escaping
private static final String[][] RESULT = {
{"a", "b", "c", "d"},
{"a", "b", "1 2"},
{"foo baar", "b", ""},
{"foo\n,,\n\",,\n\"", "d", "e"}
};
@Test
public void testGetLine() throws IOException {
final CSVParser parser = CSVParser.parse(CSVINPUT, CSVFormat.DEFAULT.withIgnoreSurroundingSpaces(true));
for (final String[] re : RESULT) {
assertArrayEquals(re, parser.nextRecord().values());
}
assertNull(parser.nextRecord());
}
@Test
public void testGetRecords() throws IOException {
final CSVParser parser = CSVParser.parse(CSVINPUT, CSVFormat.DEFAULT.withIgnoreSurroundingSpaces(true));
final List<CSVRecord> records = parser.getRecords();
assertEquals(RESULT.length, records.size());
assertTrue(records.size() > 0);
for (int i = 0; i < RESULT.length; i++) {
assertArrayEquals(RESULT[i], records.get(i).values());
}
}
@Test
public void testExcelFormat1() throws IOException {
final String code =
"value1,value2,value3,value4\r\na,b,c,d\r\n x,,,"
+ "\r\n\r\n\"\"\"hello\"\"\",\" \"\"world\"\"\",\"abc\ndef\",\r\n";
final String[][] res = {
{"value1", "value2", "value3", "value4"},
{"a", "b", "c", "d"},
{" x", "", "", ""},
{""},
{"\"hello\"", " \"world\"", "abc\ndef", ""}
};
final CSVParser parser = CSVParser.parse(code, CSVFormat.EXCEL);
final List<CSVRecord> records = parser.getRecords();
assertEquals(res.length, records.size());
assertTrue(records.size() > 0);
for (int i = 0; i < res.length; i++) {
assertArrayEquals(res[i], records.get(i).values());
}
}
@Test
public void testExcelFormat2() throws Exception {
final String code = "foo,baar\r\n\r\nhello,\r\n\r\nworld,\r\n";
final String[][] res = {
{"foo", "baar"},
{""},
{"hello", ""},
{""},
{"world", ""}
};
final CSVParser parser = CSVParser.parse(code, CSVFormat.EXCEL);
final List<CSVRecord> records = parser.getRecords();
assertEquals(res.length, records.size());
assertTrue(records.size() > 0);
for (int i = 0; i < res.length; i++) {
assertArrayEquals(res[i], records.get(i).values());
}
}
@Test
public void testEndOfFileBehaviourExcel() throws Exception {
final String[] codes = {
"hello,\r\n\r\nworld,\r\n",
"hello,\r\n\r\nworld,",
"hello,\r\n\r\nworld,\"\"\r\n",
"hello,\r\n\r\nworld,\"\"",
"hello,\r\n\r\nworld,\n",
"hello,\r\n\r\nworld,",
"hello,\r\n\r\nworld,\"\"\n",
"hello,\r\n\r\nworld,\"\""
};
final String[][] res = {
{"hello", ""},
{""}, // Excel format does not ignore empty lines
{"world", ""}
};
for (final String code : codes) {
final CSVParser parser = CSVParser.parse(code, CSVFormat.EXCEL);
final List<CSVRecord> records = parser.getRecords();
assertEquals(res.length, records.size());
assertTrue(records.size() > 0);
for (int i = 0; i < res.length; i++) {
assertArrayEquals(res[i], records.get(i).values());
}
}
}
@Test
public void testEndOfFileBehaviorCSV() throws Exception {
final String[] codes = {
"hello,\r\n\r\nworld,\r\n",
"hello,\r\n\r\nworld,",
"hello,\r\n\r\nworld,\"\"\r\n",
"hello,\r\n\r\nworld,\"\"",
"hello,\r\n\r\nworld,\n",
"hello,\r\n\r\nworld,",
"hello,\r\n\r\nworld,\"\"\n",
"hello,\r\n\r\nworld,\"\""
};
final String[][] res = {
{"hello", ""}, // CSV format ignores empty lines
{"world", ""}
};
for (final String code : codes) {
final CSVParser parser = CSVParser.parse(code, CSVFormat.DEFAULT);
final List<CSVRecord> records = parser.getRecords();
assertEquals(res.length, records.size());
assertTrue(records.size() > 0);
for (int i = 0; i < res.length; i++) {
assertArrayEquals(res[i], records.get(i).values());
}
}
}
@Test
public void testEmptyLineBehaviourExcel() throws Exception {
final String[] codes = {
"hello,\r\n\r\n\r\n",
"hello,\n\n\n",
"hello,\"\"\r\n\r\n\r\n",
"hello,\"\"\n\n\n"
};
final String[][] res = {
{"hello", ""},
{""}, // Excel format does not ignore empty lines
{""}
};
for (final String code : codes) {
final CSVParser parser = CSVParser.parse(code, CSVFormat.EXCEL);
final List<CSVRecord> records = parser.getRecords();
assertEquals(res.length, records.size());
assertTrue(records.size() > 0);
for (int i = 0; i < res.length; i++) {
assertArrayEquals(res[i], records.get(i).values());
}
}
}
@Test
public void testEmptyLineBehaviourCSV() throws Exception {
final String[] codes = {
"hello,\r\n\r\n\r\n",
"hello,\n\n\n",
"hello,\"\"\r\n\r\n\r\n",
"hello,\"\"\n\n\n"
};
final String[][] res = {
{"hello", ""} // CSV format ignores empty lines
};
for (final String code : codes) {
final CSVParser parser = CSVParser.parse(code, CSVFormat.DEFAULT);
final List<CSVRecord> records = parser.getRecords();
assertEquals(res.length, records.size());
assertTrue(records.size() > 0);
for (int i = 0; i < res.length; i++) {
assertArrayEquals(res[i], records.get(i).values());
}
}
}
@Test
public void testEmptyFile() throws Exception {
final CSVParser parser = CSVParser.parse("", CSVFormat.DEFAULT);
assertNull(parser.nextRecord());
}
@Test
public void testCSV57() throws Exception {
final CSVParser parser = CSVParser.parse("", CSVFormat.DEFAULT);
final List<CSVRecord> list = parser.getRecords();
assertNotNull(list);
assertEquals(0, list.size());
}
@Test
@Ignore
public void testBackslashEscapingOld() throws IOException {
final String code =
"one,two,three\n"
+ "on\\\"e,two\n"
+ "on\"e,two\n"
+ "one,\"tw\\\"o\"\n"
+ "one,\"t\\,wo\"\n"
+ "one,two,\"th,ree\"\n"
+ "\"a\\\\\"\n"
+ "a\\,b\n"
+ "\"a\\\\,b\"";
final String[][] res = {
{"one", "two", "three"},
{"on\\\"e", "two"},
{"on\"e", "two"},
{"one", "tw\"o"},
{"one", "t\\,wo"}, // backslash in quotes only escapes a delimiter (",")
{"one", "two", "th,ree"},
{"a\\\\"}, // backslash in quotes only escapes a delimiter (",")
{"a\\", "b"}, // a backslash must be returnd
{"a\\\\,b"} // backslash in quotes only escapes a delimiter (",")
};
final CSVParser parser = CSVParser.parse(code, CSVFormat.DEFAULT);
final List<CSVRecord> records = parser.getRecords();
assertEquals(res.length, records.size());
assertTrue(records.size() > 0);
for (int i = 0; i < res.length; i++) {
assertArrayEquals(res[i], records.get(i).values());
}
}
@Test
public void testBackslashEscaping() throws IOException {
// To avoid confusion over the need for escaping chars in java code,
// We will test with a forward slash as the escape char, and a single
// quote as the encapsulator.
final String code =
"one,two,three\n" // 0
+ "'',''\n" // 1) empty encapsulators
+ "/',/'\n" // 2) single encapsulators
+ "'/'','/''\n" // 3) single encapsulators encapsulated via escape
+ "'''',''''\n" // 4) single encapsulators encapsulated via doubling
+ "/,,/,\n" // 5) separator escaped
+ "//,//\n" // 6) escape escaped
+ "'//','//'\n" // 7) escape escaped in encapsulation
+ " 8 , \"quoted \"\" /\" // string\" \n" // don't eat spaces
+ "9, /\n \n" // escaped newline
+ "";
final String[][] res = {
{"one", "two", "three"}, // 0
{"", ""}, // 1
{"'", "'"}, // 2
{"'", "'"}, // 3
{"'", "'"}, // 4
{",", ","}, // 5
{"/", "/"}, // 6
{"/", "/"}, // 7
{" 8 ", " \"quoted \"\" /\" / string\" "},
{"9", " \n "},
};
final CSVFormat format = CSVFormat.newFormat(',').withQuoteChar('\'')
.withRecordSeparator(CRLF).withEscape('/').withIgnoreEmptyLines(true);
final CSVParser parser = CSVParser.parse(code, format);
final List<CSVRecord> records = parser.getRecords();
assertTrue(records.size() > 0);
Utils.compare("Records do not match expected result", res, records);
}
@Test
public void testBackslashEscaping2() throws IOException {
// To avoid confusion over the need for escaping chars in java code,
// We will test with a forward slash as the escape char, and a single
// quote as the encapsulator.
final String code = ""
+ " , , \n" // 1)
+ " \t , , \n" // 2)
+ " // , /, , /,\n" // 3)
+ "";
final String[][] res = {
{" ", " ", " "}, // 1
{" \t ", " ", " "}, // 2
{" / ", " , ", " ,"}, // 3
};
final CSVFormat format = CSVFormat.newFormat(',')
.withRecordSeparator(CRLF).withEscape('/').withIgnoreEmptyLines(true);
final CSVParser parser = CSVParser.parse(code, format);
final List<CSVRecord> records = parser.getRecords();
assertTrue(records.size() > 0);
Utils.compare("", res, records);
}
@Test
public void testDefaultFormat() throws IOException {
final String code = ""
+ "a,b#\n" // 1)
+ "\"\n\",\" \",#\n" // 2)
+ "#,\"\"\n" // 3)
+ "# Final comment\n"// 4)
;
final String[][] res = {
{"a", "b#"},
{"\n", " ", "#"},
{"#", ""},
{"# Final comment"}
};
CSVFormat format = CSVFormat.DEFAULT;
assertFalse(format.isCommentingEnabled());
CSVParser parser = CSVParser.parse(code, format);
List<CSVRecord> records = parser.getRecords();
assertTrue(records.size() > 0);
Utils.compare("Failed to parse without comments", res, records);
final String[][] res_comments = {
{"a", "b#"},
{"\n", " ", "#"},
};
format = CSVFormat.DEFAULT.withCommentStart('#');
parser = CSVParser.parse(code, format);
records = parser.getRecords();
Utils.compare("Failed to parse with comments", res_comments, records);
}
@Test
public void testCarriageReturnLineFeedEndings() throws IOException {
final String code = "foo\r\nbaar,\r\nhello,world\r\n,kanu";
final CSVParser parser = CSVParser.parse(code, CSVFormat.DEFAULT);
final List<CSVRecord> records = parser.getRecords();
assertEquals(4, records.size());
}
@Test(expected = NoSuchElementException.class)
public void testClose() throws Exception {
final Reader in = new StringReader("# comment\na,b,c\n1,2,3\nx,y,z");
final CSVParser parser = CSVFormat.DEFAULT.withCommentStart('#').withHeader().parse(in);
final Iterator<CSVRecord> records = parser.iterator();
assertTrue(records.hasNext());
parser.close();
assertFalse(records.hasNext());
records.next();
}
@Test
public void testCarriageReturnEndings() throws IOException {
final String code = "foo\rbaar,\rhello,world\r,kanu";
final CSVParser parser = CSVParser.parse(code, CSVFormat.DEFAULT);
final List<CSVRecord> records = parser.getRecords();
assertEquals(4, records.size());
}
@Test
public void testLineFeedEndings() throws IOException {
final String code = "foo\nbaar,\nhello,world\n,kanu";
final CSVParser parser = CSVParser.parse(code, CSVFormat.DEFAULT);
final List<CSVRecord> records = parser.getRecords();
assertEquals(4, records.size());
}
@Test
public void testIgnoreEmptyLines() throws IOException {
final String code = "\nfoo,baar\n\r\n,\n\n,world\r\n\n";
//String code = "world\r\n\n";
//String code = "foo;baar\r\n\r\nhello;\r\n\r\nworld;\r\n";
final CSVParser parser = CSVParser.parse(code, CSVFormat.DEFAULT);
final List<CSVRecord> records = parser.getRecords();
assertEquals(3, records.size());
}
@Test
public void testForEach() throws Exception {
final List<CSVRecord> records = new ArrayList<CSVRecord>();
final Reader in = new StringReader("a,b,c\n1,2,3\nx,y,z");
for (final CSVRecord record : CSVFormat.DEFAULT.parse(in)) {
records.add(record);
}
assertEquals(3, records.size());
assertArrayEquals(new String[]{"a", "b", "c"}, records.get(0).values());
assertArrayEquals(new String[]{"1", "2", "3"}, records.get(1).values());
assertArrayEquals(new String[]{"x", "y", "z"}, records.get(2).values());
}
@Test
public void testRoundtrip() throws Exception {
final StringWriter out = new StringWriter();
final CSVPrinter printer = new CSVPrinter(out, CSVFormat.DEFAULT);
final String input = "a,b,c\r\n1,2,3\r\nx,y,z\r\n";
for (final CSVRecord record : CSVParser.parse(input, CSVFormat.DEFAULT)) {
printer.printRecord(record);
}
assertEquals(input, out.toString());
printer.close();
}
@Test
public void testIterator() throws Exception {
final Reader in = new StringReader("a,b,c\n1,2,3\nx,y,z");
final Iterator<CSVRecord> iterator = CSVFormat.DEFAULT.parse(in).iterator();
assertTrue(iterator.hasNext());
try {
iterator.remove();
fail("expected UnsupportedOperationException");
} catch (final UnsupportedOperationException expected) {
// expected
}
assertArrayEquals(new String[]{"a", "b", "c"}, iterator.next().values());
assertArrayEquals(new String[]{"1", "2", "3"}, iterator.next().values());
assertTrue(iterator.hasNext());
assertTrue(iterator.hasNext());
assertTrue(iterator.hasNext());
assertArrayEquals(new String[]{"x", "y", "z"}, iterator.next().values());
assertFalse(iterator.hasNext());
try {
iterator.next();
fail("NoSuchElementException expected");
} catch (final NoSuchElementException e) {
// expected
}
}
@Test // TODO this may lead to strange behavior, throw an exception if iterator() has already been called?
public void testMultipleIterators() throws Exception {
CSVParser parser = CSVParser.parse("a,b,c" + CR + "d,e,f", CSVFormat.DEFAULT);
Iterator<CSVRecord> itr1 = parser.iterator();
Iterator<CSVRecord> itr2 = parser.iterator();
CSVRecord first = itr1.next();
assertEquals("a", first.get(0));
assertEquals("b", first.get(1));
assertEquals("c", first.get(2));
CSVRecord second = itr2.next();
assertEquals("d", second.get(0));
assertEquals("e", second.get(1));
assertEquals("f", second.get(2));
}
@Test
public void testHeader() throws Exception {
final Reader in = new StringReader("a,b,c\n1,2,3\nx,y,z");
final Iterator<CSVRecord> records = CSVFormat.DEFAULT.withHeader().parse(in).iterator();
for (int i = 0; i < 2; i++) {
assertTrue(records.hasNext());
final CSVRecord record = records.next();
assertEquals(record.get(0), record.get("a"));
assertEquals(record.get(1), record.get("b"));
assertEquals(record.get(2), record.get("c"));
}
assertFalse(records.hasNext());
}
@Test
public void testSkipSetHeader() throws Exception {
final Reader in = new StringReader("a,b,c\n1,2,3\nx,y,z");
final Iterator<CSVRecord> records = CSVFormat.DEFAULT.withHeader("a", "b", "c").withSkipHeaderRecord(true)
.parse(in).iterator();
final CSVRecord record = records.next();
assertEquals("1", record.get("a"));
assertEquals("2", record.get("b"));
assertEquals("3", record.get("c"));
}
@Test
public void testSkipAutoHeader() throws Exception {
final Reader in = new StringReader("a,b,c\n1,2,3\nx,y,z");
final Iterator<CSVRecord> records = CSVFormat.DEFAULT.withHeader().parse(in).iterator();
final CSVRecord record = records.next();
assertEquals("1", record.get("a"));
assertEquals("2", record.get("b"));
assertEquals("3", record.get("c"));
}
@Test
public void testHeaderComment() throws Exception {
final Reader in = new StringReader("# comment\na,b,c\n1,2,3\nx,y,z");
final Iterator<CSVRecord> records = CSVFormat.DEFAULT.withCommentStart('#').withHeader().parse(in).iterator();
for (int i = 0; i < 2; i++) {
assertTrue(records.hasNext());
final CSVRecord record = records.next();
assertEquals(record.get(0), record.get("a"));
assertEquals(record.get(1), record.get("b"));
assertEquals(record.get(2), record.get("c"));
}
assertFalse(records.hasNext());
}
@Test
public void testProvidedHeader() throws Exception {
final Reader in = new StringReader("a,b,c\n1,2,3\nx,y,z");
final Iterator<CSVRecord> records = CSVFormat.DEFAULT.withHeader("A", "B", "C").parse(in).iterator();
for (int i = 0; i < 3; i++) {
assertTrue(records.hasNext());
final CSVRecord record = records.next();
assertTrue(record.isMapped("A"));
assertTrue(record.isMapped("B"));
assertTrue(record.isMapped("C"));
assertFalse(record.isMapped("NOT MAPPED"));
assertEquals(record.get(0), record.get("A"));
assertEquals(record.get(1), record.get("B"));
assertEquals(record.get(2), record.get("C"));
}
assertFalse(records.hasNext());
}
@Test
public void testProvidedHeaderAuto() throws Exception {
final Reader in = new StringReader("a,b,c\n1,2,3\nx,y,z");
final Iterator<CSVRecord> records = CSVFormat.DEFAULT.withHeader().parse(in).iterator();
for (int i = 0; i < 2; i++) {
assertTrue(records.hasNext());
final CSVRecord record = records.next();
assertTrue(record.isMapped("a"));
assertTrue(record.isMapped("b"));
assertTrue(record.isMapped("c"));
assertFalse(record.isMapped("NOT MAPPED"));
assertEquals(record.get(0), record.get("a"));
assertEquals(record.get(1), record.get("b"));
assertEquals(record.get(2), record.get("c"));
}
assertFalse(records.hasNext());
}
@Test
public void testMappedButNotSetAsOutlook2007ContactExport() throws Exception {
final Reader in = new StringReader("a,b,c\n1,2\nx,y,z");
final Iterator<CSVRecord> records = CSVFormat.DEFAULT.withHeader("A", "B", "C").withSkipHeaderRecord(true)
.parse(in).iterator();
CSVRecord record;
// 1st record
record = records.next();
assertTrue(record.isMapped("A"));
assertTrue(record.isMapped("B"));
assertTrue(record.isMapped("C"));
assertTrue(record.isSet("A"));
assertTrue(record.isSet("B"));
assertFalse(record.isSet("C"));
assertEquals("1", record.get("A"));
assertEquals("2", record.get("B"));
assertFalse(record.isConsistent());
// 2nd record
record = records.next();
assertTrue(record.isMapped("A"));
assertTrue(record.isMapped("B"));
assertTrue(record.isMapped("C"));
assertTrue(record.isSet("A"));
assertTrue(record.isSet("B"));
assertTrue(record.isSet("C"));
assertEquals("x", record.get("A"));
assertEquals("y", record.get("B"));
assertEquals("z", record.get("C"));
assertTrue(record.isConsistent());
assertFalse(records.hasNext());
}
@Test
public void testGetHeaderMap() throws Exception {
final CSVParser parser = CSVParser.parse("a,b,c\n1,2,3\nx,y,z", CSVFormat.DEFAULT.withHeader("A", "B", "C"));
final Map<String, Integer> headerMap = parser.getHeaderMap();
final Iterator<String> columnNames = headerMap.keySet().iterator();
// Headers are iterated in column order.
Assert.assertEquals("A", columnNames.next());
Assert.assertEquals("B", columnNames.next());
Assert.assertEquals("C", columnNames.next());
final Iterator<CSVRecord> records = parser.iterator();
// Parse to make sure getHeaderMap did not have a side-effect.
for (int i = 0; i < 3; i++) {
assertTrue(records.hasNext());
final CSVRecord record = records.next();
assertEquals(record.get(0), record.get("A"));
assertEquals(record.get(1), record.get("B"));
assertEquals(record.get(2), record.get("C"));
}
assertFalse(records.hasNext());
}
@Test
public void testNoHeaderMap() throws Exception {
final CSVParser parser = CSVParser.parse("a,b,c\n1,2,3\nx,y,z", CSVFormat.DEFAULT);
Assert.assertNull(parser.getHeaderMap());
}
@Test
public void testGetLineNumberWithLF() throws Exception {
this.validateLineNumbers(String.valueOf(LF));
}
@Test
public void testGetLineNumberWithCRLF() throws Exception {
this.validateLineNumbers(CRLF);
}
@Test
public void testGetLineNumberWithCR() throws Exception {
this.validateLineNumbers(String.valueOf(CR));
}
@Test
public void testGetRecordNumberWithLF() throws Exception {
this.validateRecordNumbers(String.valueOf(LF));
}
@Test
public void testGetRecordWithMultiLineValues() throws Exception {
final CSVParser parser = CSVParser.parse("\"a\r\n1\",\"a\r\n2\"" + CRLF + "\"b\r\n1\",\"b\r\n2\"" + CRLF + "\"c\r\n1\",\"c\r\n2\"",
CSVFormat.DEFAULT.withRecordSeparator(CRLF));
CSVRecord record;
assertEquals(0, parser.getRecordNumber());
assertEquals(0, parser.getCurrentLineNumber());
assertNotNull(record = parser.nextRecord());
assertEquals(3, parser.getCurrentLineNumber());
assertEquals(1, record.getRecordNumber());
assertEquals(1, parser.getRecordNumber());
assertNotNull(record = parser.nextRecord());
assertEquals(6, parser.getCurrentLineNumber());
assertEquals(2, record.getRecordNumber());
assertEquals(2, parser.getRecordNumber());
assertNotNull(record = parser.nextRecord());
assertEquals(8, parser.getCurrentLineNumber());
assertEquals(3, record.getRecordNumber());
assertEquals(3, parser.getRecordNumber());
assertNull(record = parser.nextRecord());
assertEquals(8, parser.getCurrentLineNumber());
assertEquals(3, parser.getRecordNumber());
}
@Test
public void testGetRecordNumberWithCRLF() throws Exception {
this.validateRecordNumbers(CRLF);
}
@Test
public void testGetRecordNumberWithCR() throws Exception {
this.validateRecordNumbers(String.valueOf(CR));
}
@Test(expected = IllegalArgumentException.class)
public void testInvalidFormat() throws Exception {
final CSVFormat invalidFormat = CSVFormat.DEFAULT.withDelimiter(CR);
new CSVParser(null, invalidFormat).close();
}
@Test(expected = IllegalArgumentException.class)
public void testParseNullFileFormat() throws Exception {
CSVParser.parse((File) null, CSVFormat.DEFAULT);
}
@Test(expected = IllegalArgumentException.class)
public void testParseFileNullFormat() throws Exception {
CSVParser.parse(new File(""), null);
}
@Test(expected = IllegalArgumentException.class)
public void testParseNullStringFormat() throws Exception {
CSVParser.parse((String) null, CSVFormat.DEFAULT);
}
@Test(expected = IllegalArgumentException.class)
public void testParseStringNullFormat() throws Exception {
CSVParser.parse("csv data", null);
}
@Test(expected = IllegalArgumentException.class)
public void testParseNullUrlCharsetFormat() throws Exception {
CSVParser.parse(null, Charset.defaultCharset(), CSVFormat.DEFAULT);
}
@Test(expected = IllegalArgumentException.class)
public void testParserUrlNullCharsetFormat() throws Exception {
CSVParser.parse(new URL("http://commons.apache.org"), null, CSVFormat.DEFAULT);
}
@Test(expected = IllegalArgumentException.class)
public void testParseUrlCharsetNullFormat() throws Exception {
CSVParser.parse(new URL("http://commons.apache.org"), Charset.defaultCharset(), null);
}
@Test(expected = IllegalArgumentException.class)
public void testNewCSVParserNullReaderFormat() throws Exception {
new CSVParser(null, CSVFormat.DEFAULT);
}
@Test(expected = IllegalArgumentException.class)
public void testNewCSVParserReaderNullFormat() throws Exception {
new CSVParser(new StringReader(""), null);
}
private void validateRecordNumbers(final String lineSeparator) throws IOException {
final CSVParser parser = CSVParser.parse("a" + lineSeparator + "b" + lineSeparator + "c", CSVFormat.DEFAULT.withRecordSeparator(lineSeparator));
CSVRecord record;
assertEquals(0, parser.getRecordNumber());
assertNotNull(record = parser.nextRecord());
assertEquals(1, record.getRecordNumber());
assertEquals(1, parser.getRecordNumber());
assertNotNull(record = parser.nextRecord());
assertEquals(2, record.getRecordNumber());
assertEquals(2, parser.getRecordNumber());
assertNotNull(record = parser.nextRecord());
assertEquals(3, record.getRecordNumber());
assertEquals(3, parser.getRecordNumber());
assertNull(record = parser.nextRecord());
assertEquals(3, parser.getRecordNumber());
}
private void validateLineNumbers(final String lineSeparator) throws IOException {
final CSVParser parser = CSVParser.parse("a" + lineSeparator + "b" + lineSeparator + "c", CSVFormat.DEFAULT.withRecordSeparator(lineSeparator));
assertEquals(0, parser.getCurrentLineNumber());
assertNotNull(parser.nextRecord());
assertEquals(1, parser.getCurrentLineNumber());
assertNotNull(parser.nextRecord());
assertEquals(2, parser.getCurrentLineNumber());
assertNotNull(parser.nextRecord());
// Still 2 because the last line is does not have EOL chars
assertEquals(2, parser.getCurrentLineNumber());
assertNull(parser.nextRecord());
// Still 2 because the last line is does not have EOL chars
assertEquals(2, parser.getCurrentLineNumber());
}
} |
||
public void testEmpty() {
TextBuffer tb = new TextBuffer(new BufferRecycler());
tb.resetWithEmpty();
assertTrue(tb.getTextBuffer().length == 0);
tb.contentsAsString();
assertTrue(tb.getTextBuffer().length == 0);
} | com.fasterxml.jackson.core.util.TestTextBuffer::testEmpty | src/test/java/com/fasterxml/jackson/core/util/TestTextBuffer.java | 85 | src/test/java/com/fasterxml/jackson/core/util/TestTextBuffer.java | testEmpty | package com.fasterxml.jackson.core.util;
public class TestTextBuffer
extends com.fasterxml.jackson.core.BaseTest
{
/**
* Trivially simple basic test to ensure all basic append
* methods work
*/
public void testSimple()
{
TextBuffer tb = new TextBuffer(new BufferRecycler());
tb.append('a');
tb.append(new char[] { 'X', 'b' }, 1, 1);
tb.append("c", 0, 1);
assertEquals(3, tb.contentsAsArray().length);
assertEquals("abc", tb.toString());
assertNotNull(tb.expandCurrentSegment());
}
public void testLonger()
{
TextBuffer tb = new TextBuffer(new BufferRecycler());
for (int i = 0; i < 2000; ++i) {
tb.append("abc", 0, 3);
}
String str = tb.contentsAsString();
assertEquals(6000, str.length());
assertEquals(6000, tb.contentsAsArray().length);
tb.resetWithShared(new char[] { 'a' }, 0, 1);
assertEquals(1, tb.toString().length());
}
public void testLongAppend()
{
final int len = TextBuffer.MAX_SEGMENT_LEN * 3 / 2;
StringBuilder sb = new StringBuilder(len);
for (int i = 0; i < len; ++i) {
sb.append('x');
}
final String STR = sb.toString();
final String EXP = "a" + STR + "c";
// ok: first test with String:
TextBuffer tb = new TextBuffer(new BufferRecycler());
tb.append('a');
tb.append(STR, 0, len);
tb.append('c');
assertEquals(len+2, tb.size());
assertEquals(EXP, tb.contentsAsString());
// then char[]
tb = new TextBuffer(new BufferRecycler());
tb.append('a');
tb.append(STR.toCharArray(), 0, len);
tb.append('c');
assertEquals(len+2, tb.size());
assertEquals(EXP, tb.contentsAsString());
}
// [Core#152]
public void testExpand()
{
TextBuffer tb = new TextBuffer(new BufferRecycler());
char[] buf = tb.getCurrentSegment();
while (buf.length < 500 * 1000) {
char[] old = buf;
buf = tb.expandCurrentSegment();
if (old.length >= buf.length) {
fail("Expected buffer of "+old.length+" to expand, did not, length now "+buf.length);
}
}
}
// [Core#182]
public void testEmpty() {
TextBuffer tb = new TextBuffer(new BufferRecycler());
tb.resetWithEmpty();
assertTrue(tb.getTextBuffer().length == 0);
tb.contentsAsString();
assertTrue(tb.getTextBuffer().length == 0);
}
} | // You are a professional Java test case writer, please create a test case named `testEmpty` for the issue `JacksonCore-182`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonCore-182
//
// ## Issue-Title:
// Inconsistent TextBuffer#getTextBuffer behavior
//
// ## Issue-Description:
// Hi, I'm using 2.4.2. While I'm working on CBORParser, I noticed that CBORParser#getTextCharacters() returns sometimes `null` sometimes `[]` (empty array) when it's parsing empty string `""`.
//
//
// While debugging, I noticed that TextBuffer#getTextBuffer behaves inconsistently.
//
//
//
// ```
// TextBuffer buffer = new TextBuffer(new BufferRecycler());
// buffer.resetWithEmpty();
// buffer.getTextBuffer(); // returns null
// buffer.contentsAsString(); // returns empty string ""
// buffer.getTextBuffer(); // returns empty array []
//
// ```
//
// I think getTextBuffer should return the same value. Not sure which (`null` or `[]`) is expected though.
//
//
//
//
public void testEmpty() {
| 85 | // [Core#182] | 8 | 78 | src/test/java/com/fasterxml/jackson/core/util/TestTextBuffer.java | src/test/java | ```markdown
## Issue-ID: JacksonCore-182
## Issue-Title:
Inconsistent TextBuffer#getTextBuffer behavior
## Issue-Description:
Hi, I'm using 2.4.2. While I'm working on CBORParser, I noticed that CBORParser#getTextCharacters() returns sometimes `null` sometimes `[]` (empty array) when it's parsing empty string `""`.
While debugging, I noticed that TextBuffer#getTextBuffer behaves inconsistently.
```
TextBuffer buffer = new TextBuffer(new BufferRecycler());
buffer.resetWithEmpty();
buffer.getTextBuffer(); // returns null
buffer.contentsAsString(); // returns empty string ""
buffer.getTextBuffer(); // returns empty array []
```
I think getTextBuffer should return the same value. Not sure which (`null` or `[]`) is expected though.
```
You are a professional Java test case writer, please create a test case named `testEmpty` for the issue `JacksonCore-182`, utilizing the provided issue report information and the following function signature.
```java
public void testEmpty() {
```
| 78 | [
"com.fasterxml.jackson.core.util.TextBuffer"
] | 8e084a02916664f23ad6f54f1ea1c573ff7eabc3b9da922f38ce7ed21ede57ae | public void testEmpty() | // You are a professional Java test case writer, please create a test case named `testEmpty` for the issue `JacksonCore-182`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonCore-182
//
// ## Issue-Title:
// Inconsistent TextBuffer#getTextBuffer behavior
//
// ## Issue-Description:
// Hi, I'm using 2.4.2. While I'm working on CBORParser, I noticed that CBORParser#getTextCharacters() returns sometimes `null` sometimes `[]` (empty array) when it's parsing empty string `""`.
//
//
// While debugging, I noticed that TextBuffer#getTextBuffer behaves inconsistently.
//
//
//
// ```
// TextBuffer buffer = new TextBuffer(new BufferRecycler());
// buffer.resetWithEmpty();
// buffer.getTextBuffer(); // returns null
// buffer.contentsAsString(); // returns empty string ""
// buffer.getTextBuffer(); // returns empty array []
//
// ```
//
// I think getTextBuffer should return the same value. Not sure which (`null` or `[]`) is expected though.
//
//
//
//
| JacksonCore | package com.fasterxml.jackson.core.util;
public class TestTextBuffer
extends com.fasterxml.jackson.core.BaseTest
{
/**
* Trivially simple basic test to ensure all basic append
* methods work
*/
public void testSimple()
{
TextBuffer tb = new TextBuffer(new BufferRecycler());
tb.append('a');
tb.append(new char[] { 'X', 'b' }, 1, 1);
tb.append("c", 0, 1);
assertEquals(3, tb.contentsAsArray().length);
assertEquals("abc", tb.toString());
assertNotNull(tb.expandCurrentSegment());
}
public void testLonger()
{
TextBuffer tb = new TextBuffer(new BufferRecycler());
for (int i = 0; i < 2000; ++i) {
tb.append("abc", 0, 3);
}
String str = tb.contentsAsString();
assertEquals(6000, str.length());
assertEquals(6000, tb.contentsAsArray().length);
tb.resetWithShared(new char[] { 'a' }, 0, 1);
assertEquals(1, tb.toString().length());
}
public void testLongAppend()
{
final int len = TextBuffer.MAX_SEGMENT_LEN * 3 / 2;
StringBuilder sb = new StringBuilder(len);
for (int i = 0; i < len; ++i) {
sb.append('x');
}
final String STR = sb.toString();
final String EXP = "a" + STR + "c";
// ok: first test with String:
TextBuffer tb = new TextBuffer(new BufferRecycler());
tb.append('a');
tb.append(STR, 0, len);
tb.append('c');
assertEquals(len+2, tb.size());
assertEquals(EXP, tb.contentsAsString());
// then char[]
tb = new TextBuffer(new BufferRecycler());
tb.append('a');
tb.append(STR.toCharArray(), 0, len);
tb.append('c');
assertEquals(len+2, tb.size());
assertEquals(EXP, tb.contentsAsString());
}
// [Core#152]
public void testExpand()
{
TextBuffer tb = new TextBuffer(new BufferRecycler());
char[] buf = tb.getCurrentSegment();
while (buf.length < 500 * 1000) {
char[] old = buf;
buf = tb.expandCurrentSegment();
if (old.length >= buf.length) {
fail("Expected buffer of "+old.length+" to expand, did not, length now "+buf.length);
}
}
}
// [Core#182]
public void testEmpty() {
TextBuffer tb = new TextBuffer(new BufferRecycler());
tb.resetWithEmpty();
assertTrue(tb.getTextBuffer().length == 0);
tb.contentsAsString();
assertTrue(tb.getTextBuffer().length == 0);
}
} |
|
public void testStripLeadingAndTrailingQuotes()
{
assertEquals("foo", Util.stripLeadingAndTrailingQuotes("\"foo\""));
assertEquals("foo \"bar\"", Util.stripLeadingAndTrailingQuotes("foo \"bar\""));
assertEquals("\"foo\" bar", Util.stripLeadingAndTrailingQuotes("\"foo\" bar"));
assertEquals("\"foo\" and \"bar\"", Util.stripLeadingAndTrailingQuotes("\"foo\" and \"bar\""));
assertEquals("\"", Util.stripLeadingAndTrailingQuotes("\""));
} | org.apache.commons.cli.UtilTest::testStripLeadingAndTrailingQuotes | src/test/org/apache/commons/cli/UtilTest.java | 41 | src/test/org/apache/commons/cli/UtilTest.java | testStripLeadingAndTrailingQuotes | /**
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.cli;
import junit.framework.TestCase;
/**
* @author brianegge
*/
public class UtilTest extends TestCase
{
public void testStripLeadingHyphens()
{
assertEquals("f", Util.stripLeadingHyphens("-f"));
assertEquals("foo", Util.stripLeadingHyphens("--foo"));
assertEquals("-foo", Util.stripLeadingHyphens("---foo"));
assertNull(Util.stripLeadingHyphens(null));
}
public void testStripLeadingAndTrailingQuotes()
{
assertEquals("foo", Util.stripLeadingAndTrailingQuotes("\"foo\""));
assertEquals("foo \"bar\"", Util.stripLeadingAndTrailingQuotes("foo \"bar\""));
assertEquals("\"foo\" bar", Util.stripLeadingAndTrailingQuotes("\"foo\" bar"));
assertEquals("\"foo\" and \"bar\"", Util.stripLeadingAndTrailingQuotes("\"foo\" and \"bar\""));
assertEquals("\"", Util.stripLeadingAndTrailingQuotes("\""));
}
} | // You are a professional Java test case writer, please create a test case named `testStripLeadingAndTrailingQuotes` for the issue `Cli-CLI-185`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Cli-CLI-185
//
// ## Issue-Title:
// Commons CLI incorrectly stripping leading and trailing quotes
//
// ## Issue-Description:
//
// org.apache.commons.cli.Parser.processArgs() calls Util.stripLeadingAndTrailingQuotes() for all argument values. IMHO this is incorrect and totally broken.
//
//
// It is trivial to create a simple test for this. Output:
//
//
// $ java -cp target/clitest.jar Clitest --balloo "this is a \"test\""
//
// Value of argument balloo is 'this is a "test'.
//
//
// The argument 'balloo' should indeed keep its trailing double quote. It is what the shell gives it, so don't try to do something clever to it.
//
//
// The offending code was committed here:
//
// <http://svn.apache.org/viewvc?view=rev&revision=129874>
//
// and has been there for more than 6 years . Why was this committed in the first place?
//
//
// The fix is trivial, just get rid of Util.stripLeadingAndTrailingQuotes(), and consequently avoid calling it from Parser.processArgs().
//
//
//
//
//
public void testStripLeadingAndTrailingQuotes() {
| 41 | 29 | 34 | src/test/org/apache/commons/cli/UtilTest.java | src/test | ```markdown
## Issue-ID: Cli-CLI-185
## Issue-Title:
Commons CLI incorrectly stripping leading and trailing quotes
## Issue-Description:
org.apache.commons.cli.Parser.processArgs() calls Util.stripLeadingAndTrailingQuotes() for all argument values. IMHO this is incorrect and totally broken.
It is trivial to create a simple test for this. Output:
$ java -cp target/clitest.jar Clitest --balloo "this is a \"test\""
Value of argument balloo is 'this is a "test'.
The argument 'balloo' should indeed keep its trailing double quote. It is what the shell gives it, so don't try to do something clever to it.
The offending code was committed here:
<http://svn.apache.org/viewvc?view=rev&revision=129874>
and has been there for more than 6 years . Why was this committed in the first place?
The fix is trivial, just get rid of Util.stripLeadingAndTrailingQuotes(), and consequently avoid calling it from Parser.processArgs().
```
You are a professional Java test case writer, please create a test case named `testStripLeadingAndTrailingQuotes` for the issue `Cli-CLI-185`, utilizing the provided issue report information and the following function signature.
```java
public void testStripLeadingAndTrailingQuotes() {
```
| 34 | [
"org.apache.commons.cli.Util"
] | 8e4dc96b9f856e20500c0993b9056f8f0d7b0d54e67e7e65373455578f6dd9b5 | public void testStripLeadingAndTrailingQuotes()
| // You are a professional Java test case writer, please create a test case named `testStripLeadingAndTrailingQuotes` for the issue `Cli-CLI-185`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Cli-CLI-185
//
// ## Issue-Title:
// Commons CLI incorrectly stripping leading and trailing quotes
//
// ## Issue-Description:
//
// org.apache.commons.cli.Parser.processArgs() calls Util.stripLeadingAndTrailingQuotes() for all argument values. IMHO this is incorrect and totally broken.
//
//
// It is trivial to create a simple test for this. Output:
//
//
// $ java -cp target/clitest.jar Clitest --balloo "this is a \"test\""
//
// Value of argument balloo is 'this is a "test'.
//
//
// The argument 'balloo' should indeed keep its trailing double quote. It is what the shell gives it, so don't try to do something clever to it.
//
//
// The offending code was committed here:
//
// <http://svn.apache.org/viewvc?view=rev&revision=129874>
//
// and has been there for more than 6 years . Why was this committed in the first place?
//
//
// The fix is trivial, just get rid of Util.stripLeadingAndTrailingQuotes(), and consequently avoid calling it from Parser.processArgs().
//
//
//
//
//
| Cli | /**
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.cli;
import junit.framework.TestCase;
/**
* @author brianegge
*/
public class UtilTest extends TestCase
{
public void testStripLeadingHyphens()
{
assertEquals("f", Util.stripLeadingHyphens("-f"));
assertEquals("foo", Util.stripLeadingHyphens("--foo"));
assertEquals("-foo", Util.stripLeadingHyphens("---foo"));
assertNull(Util.stripLeadingHyphens(null));
}
public void testStripLeadingAndTrailingQuotes()
{
assertEquals("foo", Util.stripLeadingAndTrailingQuotes("\"foo\""));
assertEquals("foo \"bar\"", Util.stripLeadingAndTrailingQuotes("foo \"bar\""));
assertEquals("\"foo\" bar", Util.stripLeadingAndTrailingQuotes("\"foo\" bar"));
assertEquals("\"foo\" and \"bar\"", Util.stripLeadingAndTrailingQuotes("\"foo\" and \"bar\""));
assertEquals("\"", Util.stripLeadingAndTrailingQuotes("\""));
}
} |
||
public void testNumericKeys() {
assertPrint("var x = {010: 1};", "var x={8:1}");
assertPrint("var x = {'010': 1};", "var x={\"010\":1}");
assertPrint("var x = {0x10: 1};", "var x={16:1}");
assertPrint("var x = {'0x10': 1};", "var x={\"0x10\":1}");
// I was surprised at this result too.
assertPrint("var x = {.2: 1};", "var x={\"0.2\":1}");
assertPrint("var x = {'.2': 1};", "var x={\".2\":1}");
assertPrint("var x = {0.2: 1};", "var x={\"0.2\":1}");
assertPrint("var x = {'0.2': 1};", "var x={\"0.2\":1}");
} | com.google.javascript.jscomp.CodePrinterTest::testNumericKeys | test/com/google/javascript/jscomp/CodePrinterTest.java | 1,269 | test/com/google/javascript/jscomp/CodePrinterTest.java | testNumericKeys | /*
* Copyright 2004 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import com.google.javascript.jscomp.CompilerOptions.LanguageMode;
import com.google.javascript.rhino.InputId;
import com.google.javascript.rhino.Node;
import com.google.javascript.rhino.Token;
import junit.framework.TestCase;
public class CodePrinterTest extends TestCase {
static Node parse(String js) {
return parse(js, false);
}
static Node parse(String js, boolean checkTypes) {
Compiler compiler = new Compiler();
CompilerOptions options = new CompilerOptions();
// Allow getters and setters.
options.setLanguageIn(LanguageMode.ECMASCRIPT5);
compiler.initOptions(options);
Node n = compiler.parseTestCode(js);
if (checkTypes) {
DefaultPassConfig passConfig = new DefaultPassConfig(null);
CompilerPass typeResolver = passConfig.resolveTypes.create(compiler);
Node externs = new Node(Token.SCRIPT);
externs.setInputId(new InputId("externs"));
externs.setIsSyntheticBlock(true);
Node externAndJsRoot = new Node(Token.BLOCK, externs, n);
externAndJsRoot.setIsSyntheticBlock(true);
typeResolver.process(externs, n);
CompilerPass inferTypes = passConfig.inferTypes.create(compiler);
inferTypes.process(externs, n);
}
checkUnexpectedErrorsOrWarnings(compiler, 0);
return n;
}
private static void checkUnexpectedErrorsOrWarnings(
Compiler compiler, int expected) {
int actual = compiler.getErrors().length + compiler.getWarnings().length;
if (actual != expected) {
String msg = "";
for (JSError err : compiler.getErrors()) {
msg += "Error:" + err.toString() + "\n";
}
for (JSError err : compiler.getWarnings()) {
msg += "Warning:" + err.toString() + "\n";
}
assertEquals("Unexpected warnings or errors.\n " + msg, expected, actual);
}
}
String parsePrint(String js, boolean prettyprint, int lineThreshold) {
return new CodePrinter.Builder(parse(js)).setPrettyPrint(prettyprint)
.setLineLengthThreshold(lineThreshold).build();
}
String parsePrint(String js, boolean prettyprint, boolean lineBreak,
int lineThreshold) {
return new CodePrinter.Builder(parse(js)).setPrettyPrint(prettyprint)
.setLineLengthThreshold(lineThreshold).setLineBreak(lineBreak).build();
}
String parsePrint(String js, boolean prettyprint, boolean lineBreak,
int lineThreshold, boolean outputTypes) {
return new CodePrinter.Builder(parse(js, true)).setPrettyPrint(prettyprint)
.setOutputTypes(outputTypes)
.setLineLengthThreshold(lineThreshold).setLineBreak(lineBreak)
.build();
}
String parsePrint(String js, boolean prettyprint, boolean lineBreak,
int lineThreshold, boolean outputTypes,
boolean tagAsStrict) {
return new CodePrinter.Builder(parse(js, true)).setPrettyPrint(prettyprint)
.setOutputTypes(outputTypes)
.setLineLengthThreshold(lineThreshold).setLineBreak(lineBreak)
.setTagAsStrict(tagAsStrict)
.build();
}
String printNode(Node n) {
return new CodePrinter.Builder(n).setLineLengthThreshold(
CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD).build();
}
void assertPrintNode(String expectedJs, Node ast) {
assertEquals(expectedJs, printNode(ast));
}
public void testPrint() {
assertPrint("10 + a + b", "10+a+b");
assertPrint("10 + (30*50)", "10+30*50");
assertPrint("with(x) { x + 3; }", "with(x)x+3");
assertPrint("\"aa'a\"", "\"aa'a\"");
assertPrint("\"aa\\\"a\"", "'aa\"a'");
assertPrint("function foo()\n{return 10;}", "function foo(){return 10}");
assertPrint("a instanceof b", "a instanceof b");
assertPrint("typeof(a)", "typeof a");
assertPrint(
"var foo = x ? { a : 1 } : {a: 3, b:4, \"default\": 5, \"foo-bar\": 6}",
"var foo=x?{a:1}:{a:3,b:4,\"default\":5,\"foo-bar\":6}");
// Safari: needs ';' at the end of a throw statement
assertPrint("function foo(){throw 'error';}",
"function foo(){throw\"error\";}");
// Safari 3 needs a "{" around a single function
assertPrint("if (true) function foo(){return}",
"if(true){function foo(){return}}");
assertPrint("var x = 10; { var y = 20; }", "var x=10;var y=20");
assertPrint("while (x-- > 0);", "while(x-- >0);");
assertPrint("x-- >> 1", "x-- >>1");
assertPrint("(function () {})(); ",
"(function(){})()");
// Associativity
assertPrint("var a,b,c,d;a || (b&& c) && (a || d)",
"var a,b,c,d;a||b&&c&&(a||d)");
assertPrint("var a,b,c; a || (b || c); a * (b * c); a | (b | c)",
"var a,b,c;a||b||c;a*b*c;a|b|c");
assertPrint("var a,b,c; a / b / c;a / (b / c); a - (b - c);",
"var a,b,c;a/b/c;a/(b/c);a-(b-c)");
assertPrint("var a,b; a = b = 3;",
"var a,b;a=b=3");
assertPrint("var a,b,c,d; a = (b = c = (d = 3));",
"var a,b,c,d;a=b=c=d=3");
assertPrint("var a,b,c; a += (b = c += 3);",
"var a,b,c;a+=b=c+=3");
assertPrint("var a,b,c; a *= (b -= c);",
"var a,b,c;a*=b-=c");
// Break scripts
assertPrint("'<script>'", "\"<script>\"");
assertPrint("'</script>'", "\"<\\/script>\"");
assertPrint("\"</script> </SCRIPT>\"", "\"<\\/script> <\\/SCRIPT>\"");
assertPrint("'-->'", "\"--\\>\"");
assertPrint("']]>'", "\"]]\\>\"");
assertPrint("' --></script>'", "\" --\\><\\/script>\"");
assertPrint("/--> <\\/script>/g", "/--\\> <\\/script>/g");
// Break HTML start comments. Certain versions of Webkit
// begin an HTML comment when they see this.
assertPrint("'<!-- I am a string -->'", "\"<\\!-- I am a string --\\>\"");
// Precedence
assertPrint("a ? delete b[0] : 3", "a?delete b[0]:3");
assertPrint("(delete a[0])/10", "delete a[0]/10");
// optional '()' for new
// simple new
assertPrint("new A", "new A");
assertPrint("new A()", "new A");
assertPrint("new A('x')", "new A(\"x\")");
// calling instance method directly after new
assertPrint("new A().a()", "(new A).a()");
assertPrint("(new A).a()", "(new A).a()");
// this case should be fixed
assertPrint("new A('y').a()", "(new A(\"y\")).a()");
// internal class
assertPrint("new A.B", "new A.B");
assertPrint("new A.B()", "new A.B");
assertPrint("new A.B('z')", "new A.B(\"z\")");
// calling instance method directly after new internal class
assertPrint("(new A.B).a()", "(new A.B).a()");
assertPrint("new A.B().a()", "(new A.B).a()");
// this case should be fixed
assertPrint("new A.B('w').a()", "(new A.B(\"w\")).a()");
// Operators: make sure we don't convert binary + and unary + into ++
assertPrint("x + +y", "x+ +y");
assertPrint("x - (-y)", "x- -y");
assertPrint("x++ +y", "x++ +y");
assertPrint("x-- -y", "x-- -y");
assertPrint("x++ -y", "x++-y");
// Label
assertPrint("foo:for(;;){break foo;}", "foo:for(;;)break foo");
assertPrint("foo:while(1){continue foo;}", "foo:while(1)continue foo");
// Object literals.
assertPrint("({})", "({})");
assertPrint("var x = {};", "var x={}");
assertPrint("({}).x", "({}).x");
assertPrint("({})['x']", "({})[\"x\"]");
assertPrint("({}) instanceof Object", "({})instanceof Object");
assertPrint("({}) || 1", "({})||1");
assertPrint("1 || ({})", "1||{}");
assertPrint("({}) ? 1 : 2", "({})?1:2");
assertPrint("0 ? ({}) : 2", "0?{}:2");
assertPrint("0 ? 1 : ({})", "0?1:{}");
assertPrint("typeof ({})", "typeof{}");
assertPrint("f({})", "f({})");
// Anonymous function expressions.
assertPrint("(function(){})", "(function(){})");
assertPrint("(function(){})()", "(function(){})()");
assertPrint("(function(){})instanceof Object",
"(function(){})instanceof Object");
assertPrint("(function(){}).bind().call()",
"(function(){}).bind().call()");
assertPrint("var x = function() { };", "var x=function(){}");
assertPrint("var x = function() { }();", "var x=function(){}()");
assertPrint("(function() {}), 2", "(function(){}),2");
// Name functions expression.
assertPrint("(function f(){})", "(function f(){})");
// Function declaration.
assertPrint("function f(){}", "function f(){}");
// Make sure we don't treat non-latin character escapes as raw strings.
assertPrint("({ 'a': 4, '\\u0100': 4 })", "({\"a\":4,\"\\u0100\":4})");
assertPrint("({ a: 4, '\\u0100': 4 })", "({a:4,\"\\u0100\":4})");
// Test if statement and for statements with single statements in body.
assertPrint("if (true) { alert();}", "if(true)alert()");
assertPrint("if (false) {} else {alert(\"a\");}",
"if(false);else alert(\"a\")");
assertPrint("for(;;) { alert();};", "for(;;)alert()");
assertPrint("do { alert(); } while(true);",
"do alert();while(true)");
assertPrint("myLabel: { alert();}",
"myLabel:alert()");
assertPrint("myLabel: for(;;) continue myLabel;",
"myLabel:for(;;)continue myLabel");
// Test nested var statement
assertPrint("if (true) var x; x = 4;", "if(true)var x;x=4");
// Non-latin identifier. Make sure we keep them escaped.
assertPrint("\\u00fb", "\\u00fb");
assertPrint("\\u00fa=1", "\\u00fa=1");
assertPrint("function \\u00f9(){}", "function \\u00f9(){}");
assertPrint("x.\\u00f8", "x.\\u00f8");
assertPrint("x.\\u00f8", "x.\\u00f8");
assertPrint("abc\\u4e00\\u4e01jkl", "abc\\u4e00\\u4e01jkl");
// Test the right-associative unary operators for spurious parens
assertPrint("! ! true", "!!true");
assertPrint("!(!(true))", "!!true");
assertPrint("typeof(void(0))", "typeof void 0");
assertPrint("typeof(void(!0))", "typeof void!0");
assertPrint("+ - + + - + 3", "+-+ +-+3"); // chained unary plus/minus
assertPrint("+(--x)", "+--x");
assertPrint("-(++x)", "-++x");
// needs a space to prevent an ambiguous parse
assertPrint("-(--x)", "- --x");
assertPrint("!(~~5)", "!~~5");
assertPrint("~(a/b)", "~(a/b)");
// Preserve parens to overcome greedy binding of NEW
assertPrint("new (foo.bar()).factory(baz)", "new (foo.bar().factory)(baz)");
assertPrint("new (bar()).factory(baz)", "new (bar().factory)(baz)");
assertPrint("new (new foobar(x)).factory(baz)",
"new (new foobar(x)).factory(baz)");
// Make sure that HOOK is right associative
assertPrint("a ? b : (c ? d : e)", "a?b:c?d:e");
assertPrint("a ? (b ? c : d) : e", "a?b?c:d:e");
assertPrint("(a ? b : c) ? d : e", "(a?b:c)?d:e");
// Test nested ifs
assertPrint("if (x) if (y); else;", "if(x)if(y);else;");
// Test comma.
assertPrint("a,b,c", "a,b,c");
assertPrint("(a,b),c", "a,b,c");
assertPrint("a,(b,c)", "a,b,c");
assertPrint("x=a,b,c", "x=a,b,c");
assertPrint("x=(a,b),c", "x=(a,b),c");
assertPrint("x=a,(b,c)", "x=a,b,c");
assertPrint("x=a,y=b,z=c", "x=a,y=b,z=c");
assertPrint("x=(a,y=b,z=c)", "x=(a,y=b,z=c)");
assertPrint("x=[a,b,c,d]", "x=[a,b,c,d]");
assertPrint("x=[(a,b,c),d]", "x=[(a,b,c),d]");
assertPrint("x=[(a,(b,c)),d]", "x=[(a,b,c),d]");
assertPrint("x=[a,(b,c,d)]", "x=[a,(b,c,d)]");
assertPrint("var x=(a,b)", "var x=(a,b)");
assertPrint("var x=a,b,c", "var x=a,b,c");
assertPrint("var x=(a,b),c", "var x=(a,b),c");
assertPrint("var x=a,b=(c,d)", "var x=a,b=(c,d)");
assertPrint("foo(a,b,c,d)", "foo(a,b,c,d)");
assertPrint("foo((a,b,c),d)", "foo((a,b,c),d)");
assertPrint("foo((a,(b,c)),d)", "foo((a,b,c),d)");
assertPrint("f(a+b,(c,d,(e,f,g)))", "f(a+b,(c,d,e,f,g))");
assertPrint("({}) , 1 , 2", "({}),1,2");
assertPrint("({}) , {} , {}", "({}),{},{}");
// EMPTY nodes
assertPrint("if (x){}", "if(x);");
assertPrint("if(x);", "if(x);");
assertPrint("if(x)if(y);", "if(x)if(y);");
assertPrint("if(x){if(y);}", "if(x)if(y);");
assertPrint("if(x){if(y){};;;}", "if(x)if(y);");
assertPrint("if(x){;;function y(){};;}", "if(x){function y(){}}");
}
public void testPrintArray() {
assertPrint("[void 0, void 0]", "[void 0,void 0]");
assertPrint("[undefined, undefined]", "[undefined,undefined]");
assertPrint("[ , , , undefined]", "[,,,undefined]");
assertPrint("[ , , , 0]", "[,,,0]");
}
public void testHook() {
assertPrint("a ? b = 1 : c = 2", "a?b=1:c=2");
assertPrint("x = a ? b = 1 : c = 2", "x=a?b=1:c=2");
assertPrint("(x = a) ? b = 1 : c = 2", "(x=a)?b=1:c=2");
assertPrint("x, a ? b = 1 : c = 2", "x,a?b=1:c=2");
assertPrint("x, (a ? b = 1 : c = 2)", "x,a?b=1:c=2");
assertPrint("(x, a) ? b = 1 : c = 2", "(x,a)?b=1:c=2");
assertPrint("a ? (x, b) : c = 2", "a?(x,b):c=2");
assertPrint("a ? b = 1 : (x,c)", "a?b=1:(x,c)");
assertPrint("a ? b = 1 : c = 2 + x", "a?b=1:c=2+x");
assertPrint("(a ? b = 1 : c = 2) + x", "(a?b=1:c=2)+x");
assertPrint("a ? b = 1 : (c = 2) + x", "a?b=1:(c=2)+x");
assertPrint("a ? (b?1:2) : 3", "a?b?1:2:3");
}
public void testPrintInOperatorInForLoop() {
// Check for in expression in for's init expression.
// Check alone, with + (higher precedence), with ?: (lower precedence),
// and with conditional.
assertPrint("var a={}; for (var i = (\"length\" in a); i;) {}",
"var a={};for(var i=(\"length\"in a);i;);");
assertPrint("var a={}; for (var i = (\"length\" in a) ? 0 : 1; i;) {}",
"var a={};for(var i=(\"length\"in a)?0:1;i;);");
assertPrint("var a={}; for (var i = (\"length\" in a) + 1; i;) {}",
"var a={};for(var i=(\"length\"in a)+1;i;);");
assertPrint("var a={};for (var i = (\"length\" in a|| \"size\" in a);;);",
"var a={};for(var i=(\"length\"in a)||(\"size\"in a);;);");
assertPrint("var a={};for (var i = a || a || (\"size\" in a);;);",
"var a={};for(var i=a||a||(\"size\"in a);;);");
// Test works with unary operators and calls.
assertPrint("var a={}; for (var i = -(\"length\" in a); i;) {}",
"var a={};for(var i=-(\"length\"in a);i;);");
assertPrint("var a={};function b_(p){ return p;};" +
"for(var i=1,j=b_(\"length\" in a);;) {}",
"var a={};function b_(p){return p}" +
"for(var i=1,j=b_(\"length\"in a);;);");
// Test we correctly handle an in operator in the test clause.
assertPrint("var a={}; for (;(\"length\" in a);) {}",
"var a={};for(;\"length\"in a;);");
}
public void testLiteralProperty() {
assertPrint("(64).toString()", "(64).toString()");
}
private void assertPrint(String js, String expected) {
parse(expected); // validate the expected string is valid js
assertEquals(expected,
parsePrint(js, false, CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD));
}
// Make sure that the code generator doesn't associate an
// else clause with the wrong if clause.
public void testAmbiguousElseClauses() {
assertPrintNode("if(x)if(y);else;",
new Node(Token.IF,
Node.newString(Token.NAME, "x"),
new Node(Token.BLOCK,
new Node(Token.IF,
Node.newString(Token.NAME, "y"),
new Node(Token.BLOCK),
// ELSE clause for the inner if
new Node(Token.BLOCK)))));
assertPrintNode("if(x){if(y);}else;",
new Node(Token.IF,
Node.newString(Token.NAME, "x"),
new Node(Token.BLOCK,
new Node(Token.IF,
Node.newString(Token.NAME, "y"),
new Node(Token.BLOCK))),
// ELSE clause for the outer if
new Node(Token.BLOCK)));
assertPrintNode("if(x)if(y);else{if(z);}else;",
new Node(Token.IF,
Node.newString(Token.NAME, "x"),
new Node(Token.BLOCK,
new Node(Token.IF,
Node.newString(Token.NAME, "y"),
new Node(Token.BLOCK),
new Node(Token.BLOCK,
new Node(Token.IF,
Node.newString(Token.NAME, "z"),
new Node(Token.BLOCK))))),
// ELSE clause for the outermost if
new Node(Token.BLOCK)));
}
public void testLineBreak() {
// line break after function if in a statement context
assertLineBreak("function a() {}\n" +
"function b() {}",
"function a(){}\n" +
"function b(){}\n");
// line break after ; after a function
assertLineBreak("var a = {};\n" +
"a.foo = function () {}\n" +
"function b() {}",
"var a={};a.foo=function(){};\n" +
"function b(){}\n");
// break after comma after a function
assertLineBreak("var a = {\n" +
" b: function() {},\n" +
" c: function() {}\n" +
"};\n" +
"alert(a);",
"var a={b:function(){},\n" +
"c:function(){}};\n" +
"alert(a)");
}
private void assertLineBreak(String js, String expected) {
assertEquals(expected,
parsePrint(js, false, true,
CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD));
}
public void testPrettyPrinter() {
// Ensure that the pretty printer inserts line breaks at appropriate
// places.
assertPrettyPrint("(function(){})();","(function() {\n})();\n");
assertPrettyPrint("var a = (function() {});alert(a);",
"var a = function() {\n};\nalert(a);\n");
// Check we correctly handle putting brackets around all if clauses so
// we can put breakpoints inside statements.
assertPrettyPrint("if (1) {}",
"if(1) {\n" +
"}\n");
assertPrettyPrint("if (1) {alert(\"\");}",
"if(1) {\n" +
" alert(\"\")\n" +
"}\n");
assertPrettyPrint("if (1)alert(\"\");",
"if(1) {\n" +
" alert(\"\")\n" +
"}\n");
assertPrettyPrint("if (1) {alert();alert();}",
"if(1) {\n" +
" alert();\n" +
" alert()\n" +
"}\n");
// Don't add blocks if they weren't there already.
assertPrettyPrint("label: alert();",
"label:alert();\n");
// But if statements and loops get blocks automagically.
assertPrettyPrint("if (1) alert();",
"if(1) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint("for (;;) alert();",
"for(;;) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint("while (1) alert();",
"while(1) {\n" +
" alert()\n" +
"}\n");
// Do we put else clauses in blocks?
assertPrettyPrint("if (1) {} else {alert(a);}",
"if(1) {\n" +
"}else {\n alert(a)\n}\n");
// Do we add blocks to else clauses?
assertPrettyPrint("if (1) alert(a); else alert(b);",
"if(1) {\n" +
" alert(a)\n" +
"}else {\n" +
" alert(b)\n" +
"}\n");
// Do we put for bodies in blocks?
assertPrettyPrint("for(;;) { alert();}",
"for(;;) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint("for(;;) {}",
"for(;;) {\n" +
"}\n");
assertPrettyPrint("for(;;) { alert(); alert(); }",
"for(;;) {\n" +
" alert();\n" +
" alert()\n" +
"}\n");
// How about do loops?
assertPrettyPrint("do { alert(); } while(true);",
"do {\n" +
" alert()\n" +
"}while(true);\n");
// label?
assertPrettyPrint("myLabel: { alert();}",
"myLabel: {\n" +
" alert()\n" +
"}\n");
// Don't move the label on a loop, because then break {label} and
// continue {label} won't work.
assertPrettyPrint("myLabel: for(;;) continue myLabel;",
"myLabel:for(;;) {\n" +
" continue myLabel\n" +
"}\n");
assertPrettyPrint("var a;", "var a;\n");
}
public void testPrettyPrinter2() {
assertPrettyPrint(
"if(true) f();",
"if(true) {\n" +
" f()\n" +
"}\n");
assertPrettyPrint(
"if (true) { f() } else { g() }",
"if(true) {\n" +
" f()\n" +
"}else {\n" +
" g()\n" +
"}\n");
assertPrettyPrint(
"if(true) f(); for(;;) g();",
"if(true) {\n" +
" f()\n" +
"}\n" +
"for(;;) {\n" +
" g()\n" +
"}\n");
}
public void testPrettyPrinter3() {
assertPrettyPrint(
"try {} catch(e) {}if (1) {alert();alert();}",
"try {\n" +
"}catch(e) {\n" +
"}\n" +
"if(1) {\n" +
" alert();\n" +
" alert()\n" +
"}\n");
assertPrettyPrint(
"try {} finally {}if (1) {alert();alert();}",
"try {\n" +
"}finally {\n" +
"}\n" +
"if(1) {\n" +
" alert();\n" +
" alert()\n" +
"}\n");
assertPrettyPrint(
"try {} catch(e) {} finally {} if (1) {alert();alert();}",
"try {\n" +
"}catch(e) {\n" +
"}finally {\n" +
"}\n" +
"if(1) {\n" +
" alert();\n" +
" alert()\n" +
"}\n");
}
public void testPrettyPrinter4() {
assertPrettyPrint(
"function f() {}if (1) {alert();}",
"function f() {\n" +
"}\n" +
"if(1) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint(
"var f = function() {};if (1) {alert();}",
"var f = function() {\n" +
"};\n" +
"if(1) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint(
"(function() {})();if (1) {alert();}",
"(function() {\n" +
"})();\n" +
"if(1) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint(
"(function() {alert();alert();})();if (1) {alert();}",
"(function() {\n" +
" alert();\n" +
" alert()\n" +
"})();\n" +
"if(1) {\n" +
" alert()\n" +
"}\n");
}
public void testTypeAnnotations() {
assertTypeAnnotations(
"/** @constructor */ function Foo(){}",
"/**\n * @return {undefined}\n * @constructor\n */\n"
+ "function Foo() {\n}\n");
}
public void testTypeAnnotationsTypeDef() {
// TODO(johnlenz): It would be nice if there were some way to preserve
// typedefs but currently they are resolved into the basic types in the
// type registry.
assertTypeAnnotations(
"/** @typedef {Array.<number>} */ goog.java.Long;\n"
+ "/** @param {!goog.java.Long} a*/\n"
+ "function f(a){};\n",
"goog.java.Long;\n"
+ "/**\n"
+ " * @param {(Array|null)} a\n"
+ " * @return {undefined}\n"
+ " */\n"
+ "function f(a) {\n}\n");
}
public void testTypeAnnotationsAssign() {
assertTypeAnnotations("/** @constructor */ var Foo = function(){}",
"/**\n * @return {undefined}\n * @constructor\n */\n"
+ "var Foo = function() {\n};\n");
}
public void testTypeAnnotationsNamespace() {
assertTypeAnnotations("var a = {};"
+ "/** @constructor */ a.Foo = function(){}",
"var a = {};\n"
+ "/**\n * @return {undefined}\n * @constructor\n */\n"
+ "a.Foo = function() {\n};\n");
}
public void testTypeAnnotationsMemberSubclass() {
assertTypeAnnotations("var a = {};"
+ "/** @constructor */ a.Foo = function(){};"
+ "/** @constructor \n @extends {a.Foo} */ a.Bar = function(){}",
"var a = {};\n"
+ "/**\n * @return {undefined}\n * @constructor\n */\n"
+ "a.Foo = function() {\n};\n"
+ "/**\n * @return {undefined}\n * @extends {a.Foo}\n"
+ " * @constructor\n */\n"
+ "a.Bar = function() {\n};\n");
}
public void testTypeAnnotationsInterface() {
assertTypeAnnotations("var a = {};"
+ "/** @interface */ a.Foo = function(){};"
+ "/** @interface \n @extends {a.Foo} */ a.Bar = function(){}",
"var a = {};\n"
+ "/**\n * @interface\n */\n"
+ "a.Foo = function() {\n};\n"
+ "/**\n * @extends {a.Foo}\n"
+ " * @interface\n */\n"
+ "a.Bar = function() {\n};\n");
}
public void testTypeAnnotationsMultipleInterface() {
assertTypeAnnotations("var a = {};"
+ "/** @interface */ a.Foo1 = function(){};"
+ "/** @interface */ a.Foo2 = function(){};"
+ "/** @interface \n @extends {a.Foo1} \n @extends {a.Foo2} */"
+ "a.Bar = function(){}",
"var a = {};\n"
+ "/**\n * @interface\n */\n"
+ "a.Foo1 = function() {\n};\n"
+ "/**\n * @interface\n */\n"
+ "a.Foo2 = function() {\n};\n"
+ "/**\n * @extends {a.Foo1}\n"
+ " * @extends {a.Foo2}\n"
+ " * @interface\n */\n"
+ "a.Bar = function() {\n};\n");
}
public void testTypeAnnotationsMember() {
assertTypeAnnotations("var a = {};"
+ "/** @constructor */ a.Foo = function(){}"
+ "/** @param {string} foo\n"
+ " * @return {number} */\n"
+ "a.Foo.prototype.foo = function(foo) { return 3; };"
+ "/** @type {string|undefined} */"
+ "a.Foo.prototype.bar = '';",
"var a = {};\n"
+ "/**\n * @return {undefined}\n * @constructor\n */\n"
+ "a.Foo = function() {\n};\n"
+ "/**\n"
+ " * @param {string} foo\n"
+ " * @return {number}\n"
+ " */\n"
+ "a.Foo.prototype.foo = function(foo) {\n return 3\n};\n"
+ "/** @type {string} */\n"
+ "a.Foo.prototype.bar = \"\";\n");
}
public void testTypeAnnotationsImplements() {
assertTypeAnnotations("var a = {};"
+ "/** @constructor */ a.Foo = function(){};\n"
+ "/** @interface */ a.I = function(){};\n"
+ "/** @interface */ a.I2 = function(){};\n"
+ "/** @constructor \n @extends {a.Foo}\n"
+ " * @implements {a.I} \n @implements {a.I2}\n"
+ "*/ a.Bar = function(){}",
"var a = {};\n"
+ "/**\n * @return {undefined}\n * @constructor\n */\n"
+ "a.Foo = function() {\n};\n"
+ "/**\n * @interface\n */\n"
+ "a.I = function() {\n};\n"
+ "/**\n * @interface\n */\n"
+ "a.I2 = function() {\n};\n"
+ "/**\n * @return {undefined}\n * @extends {a.Foo}\n"
+ " * @implements {a.I}\n"
+ " * @implements {a.I2}\n * @constructor\n */\n"
+ "a.Bar = function() {\n};\n");
}
public void testTypeAnnotationsDispatcher1() {
assertTypeAnnotations(
"var a = {};\n" +
"/** \n" +
" * @constructor \n" +
" * @javadispatch \n" +
" */\n" +
"a.Foo = function(){}",
"var a = {};\n" +
"/**\n" +
" * @return {undefined}\n" +
" * @constructor\n" +
" * @javadispatch\n" +
" */\n" +
"a.Foo = function() {\n" +
"};\n");
}
public void testTypeAnnotationsDispatcher2() {
assertTypeAnnotations(
"var a = {};\n" +
"/** \n" +
" * @constructor \n" +
" */\n" +
"a.Foo = function(){}\n" +
"/**\n" +
" * @javadispatch\n" +
" */\n" +
"a.Foo.prototype.foo = function() {};",
"var a = {};\n" +
"/**\n" +
" * @return {undefined}\n" +
" * @constructor\n" +
" */\n" +
"a.Foo = function() {\n" +
"};\n" +
"/**\n" +
" * @return {undefined}\n" +
" * @javadispatch\n" +
" */\n" +
"a.Foo.prototype.foo = function() {\n" +
"};\n");
}
public void testU2UFunctionTypeAnnotation() {
assertTypeAnnotations(
"/** @type {!Function} */ var x = function() {}",
"/**\n * @constructor\n */\nvar x = function() {\n};\n");
}
public void testEmitUnknownParamTypesAsAllType() {
assertTypeAnnotations(
"var a = function(x) {}",
"/**\n" +
" * @param {*} x\n" +
" * @return {undefined}\n" +
" */\n" +
"var a = function(x) {\n};\n");
}
public void testOptionalTypesAnnotation() {
assertTypeAnnotations(
"/**\n" +
" * @param {string=} x \n" +
" */\n" +
"var a = function(x) {}",
"/**\n" +
" * @param {string=} x\n" +
" * @return {undefined}\n" +
" */\n" +
"var a = function(x) {\n};\n");
}
public void testVariableArgumentsTypesAnnotation() {
assertTypeAnnotations(
"/**\n" +
" * @param {...string} x \n" +
" */\n" +
"var a = function(x) {}",
"/**\n" +
" * @param {...string} x\n" +
" * @return {undefined}\n" +
" */\n" +
"var a = function(x) {\n};\n");
}
public void testTempConstructor() {
assertTypeAnnotations(
"var x = function() {\n/**\n * @constructor\n */\nfunction t1() {}\n" +
" /**\n * @constructor\n */\nfunction t2() {}\n" +
" t1.prototype = t2.prototype}",
"/**\n * @return {undefined}\n */\nvar x = function() {\n" +
" /**\n * @return {undefined}\n * @constructor\n */\n" +
"function t1() {\n }\n" +
" /**\n * @return {undefined}\n * @constructor\n */\n" +
"function t2() {\n }\n" +
" t1.prototype = t2.prototype\n};\n"
);
}
private void assertPrettyPrint(String js, String expected) {
assertEquals(expected,
parsePrint(js, true, false,
CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD));
}
private void assertTypeAnnotations(String js, String expected) {
assertEquals(expected,
parsePrint(js, true, false,
CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD, true));
}
public void testSubtraction() {
Compiler compiler = new Compiler();
Node n = compiler.parseTestCode("x - -4");
assertEquals(0, compiler.getErrorCount());
assertEquals(
"x- -4",
new CodePrinter.Builder(n).setLineLengthThreshold(
CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD).build());
}
public void testFunctionWithCall() {
assertPrint(
"var user = new function() {"
+ "alert(\"foo\")}",
"var user=new function(){"
+ "alert(\"foo\")}");
assertPrint(
"var user = new function() {"
+ "this.name = \"foo\";"
+ "this.local = function(){alert(this.name)};}",
"var user=new function(){"
+ "this.name=\"foo\";"
+ "this.local=function(){alert(this.name)}}");
}
public void testLineLength() {
// list
assertLineLength("var aba,bcb,cdc",
"var aba,bcb," +
"\ncdc");
// operators, and two breaks
assertLineLength(
"\"foo\"+\"bar,baz,bomb\"+\"whee\"+\";long-string\"\n+\"aaa\"",
"\"foo\"+\"bar,baz,bomb\"+" +
"\n\"whee\"+\";long-string\"+" +
"\n\"aaa\"");
// assignment
assertLineLength("var abazaba=1234",
"var abazaba=" +
"\n1234");
// statements
assertLineLength("var abab=1;var bab=2",
"var abab=1;" +
"\nvar bab=2");
// don't break regexes
assertLineLength("var a=/some[reg](ex),with.*we?rd|chars/i;var b=a",
"var a=/some[reg](ex),with.*we?rd|chars/i;" +
"\nvar b=a");
// don't break strings
assertLineLength("var a=\"foo,{bar};baz\";var b=a",
"var a=\"foo,{bar};baz\";" +
"\nvar b=a");
// don't break before post inc/dec
assertLineLength("var a=\"a\";a++;var b=\"bbb\";",
"var a=\"a\";a++;\n" +
"var b=\"bbb\"");
}
private void assertLineLength(String js, String expected) {
assertEquals(expected,
parsePrint(js, false, true, 10));
}
public void testParsePrintParse() {
testReparse("3;");
testReparse("var a = b;");
testReparse("var x, y, z;");
testReparse("try { foo() } catch(e) { bar() }");
testReparse("try { foo() } catch(e) { bar() } finally { stuff() }");
testReparse("try { foo() } finally { stuff() }");
testReparse("throw 'me'");
testReparse("function foo(a) { return a + 4; }");
testReparse("function foo() { return; }");
testReparse("var a = function(a, b) { foo(); return a + b; }");
testReparse("b = [3, 4, 'paul', \"Buchhe it\",,5];");
testReparse("v = (5, 6, 7, 8)");
testReparse("d = 34.0; x = 0; y = .3; z = -22");
testReparse("d = -x; t = !x + ~y;");
testReparse("'hi'; /* just a test */ stuff(a,b) \n" +
" foo(); // and another \n" +
" bar();");
testReparse("a = b++ + ++c; a = b++-++c; a = - --b; a = - ++b;");
testReparse("a++; b= a++; b = ++a; b = a--; b = --a; a+=2; b-=5");
testReparse("a = (2 + 3) * 4;");
testReparse("a = 1 + (2 + 3) + 4;");
testReparse("x = a ? b : c; x = a ? (b,3,5) : (foo(),bar());");
testReparse("a = b | c || d ^ e " +
"&& f & !g != h << i <= j < k >>> l > m * n % !o");
testReparse("a == b; a != b; a === b; a == b == a;" +
" (a == b) == a; a == (b == a);");
testReparse("if (a > b) a = b; if (b < 3) a = 3; else c = 4;");
testReparse("if (a == b) { a++; } if (a == 0) { a++; } else { a --; }");
testReparse("for (var i in a) b += i;");
testReparse("for (var i = 0; i < 10; i++){ b /= 2;" +
" if (b == 2)break;else continue;}");
testReparse("for (x = 0; x < 10; x++) a /= 2;");
testReparse("for (;;) a++;");
testReparse("while(true) { blah(); }while(true) blah();");
testReparse("do stuff(); while(a>b);");
testReparse("[0, null, , true, false, this];");
testReparse("s.replace(/absc/, 'X').replace(/ab/gi, 'Y');");
testReparse("new Foo; new Bar(a, b,c);");
testReparse("with(foo()) { x = z; y = t; } with(bar()) a = z;");
testReparse("delete foo['bar']; delete foo;");
testReparse("var x = { 'a':'paul', 1:'3', 2:(3,4) };");
testReparse("switch(a) { case 2: case 3: stuff(); break;" +
"case 4: morestuff(); break; default: done();}");
testReparse("x = foo['bar'] + foo['my stuff'] + foo[bar] + f.stuff;");
testReparse("a.v = b.v; x['foo'] = y['zoo'];");
testReparse("'test' in x; 3 in x; a in x;");
testReparse("'foo\"bar' + \"foo'c\" + 'stuff\\n and \\\\more'");
testReparse("x.__proto__;");
}
private void testReparse(String code) {
Compiler compiler = new Compiler();
Node parse1 = parse(code);
Node parse2 = parse(new CodePrinter.Builder(parse1).build());
String explanation = parse1.checkTreeEquals(parse2);
assertNull("\nExpected: " + compiler.toSource(parse1) +
"\nResult: " + compiler.toSource(parse2) +
"\n" + explanation, explanation);
}
public void testDoLoopIECompatiblity() {
// Do loops within IFs cause syntax errors in IE6 and IE7.
assertPrint("function f(){if(e1){do foo();while(e2)}else foo()}",
"function f(){if(e1){do foo();while(e2)}else foo()}");
assertPrint("function f(){if(e1)do foo();while(e2)else foo()}",
"function f(){if(e1){do foo();while(e2)}else foo()}");
assertPrint("if(x){do{foo()}while(y)}else bar()",
"if(x){do foo();while(y)}else bar()");
assertPrint("if(x)do{foo()}while(y);else bar()",
"if(x){do foo();while(y)}else bar()");
assertPrint("if(x){do{foo()}while(y)}",
"if(x){do foo();while(y)}");
assertPrint("if(x)do{foo()}while(y);",
"if(x){do foo();while(y)}");
assertPrint("if(x)A:do{foo()}while(y);",
"if(x){A:do foo();while(y)}");
assertPrint("var i = 0;a: do{b: do{i++;break b;} while(0);} while(0);",
"var i=0;a:do{b:do{i++;break b}while(0)}while(0)");
}
public void testFunctionSafariCompatiblity() {
// Functions within IFs cause syntax errors on Safari.
assertPrint("function f(){if(e1){function goo(){return true}}else foo()}",
"function f(){if(e1){function goo(){return true}}else foo()}");
assertPrint("function f(){if(e1)function goo(){return true}else foo()}",
"function f(){if(e1){function goo(){return true}}else foo()}");
assertPrint("if(e1){function goo(){return true}}",
"if(e1){function goo(){return true}}");
assertPrint("if(e1)function goo(){return true}",
"if(e1){function goo(){return true}}");
assertPrint("if(e1)A:function goo(){return true}",
"if(e1){A:function goo(){return true}}");
}
public void testExponents() {
assertPrintNumber("1", 1);
assertPrintNumber("10", 10);
assertPrintNumber("100", 100);
assertPrintNumber("1E3", 1000);
assertPrintNumber("1E4", 10000);
assertPrintNumber("1E5", 100000);
assertPrintNumber("-1", -1);
assertPrintNumber("-10", -10);
assertPrintNumber("-100", -100);
assertPrintNumber("-1E3", -1000);
assertPrintNumber("-12341234E4", -123412340000L);
assertPrintNumber("1E18", 1000000000000000000L);
assertPrintNumber("1E5", 100000.0);
assertPrintNumber("100000.1", 100000.1);
assertPrintNumber("1.0E-6", 0.000001);
}
// Make sure to test as both a String and a Node, because
// negative numbers do not parse consistently from strings.
private void assertPrintNumber(String expected, double number) {
assertPrint(String.valueOf(number), expected);
assertPrintNode(expected, Node.newNumber(number));
}
private void assertPrintNumber(String expected, int number) {
assertPrint(String.valueOf(number), expected);
assertPrintNode(expected, Node.newNumber(number));
}
public void testDirectEval() {
assertPrint("eval('1');", "eval(\"1\")");
}
public void testIndirectEval() {
Node n = parse("eval('1');");
assertPrintNode("eval(\"1\")", n);
n.getFirstChild().getFirstChild().getFirstChild().putBooleanProp(
Node.DIRECT_EVAL, false);
assertPrintNode("(0,eval)(\"1\")", n);
}
public void testFreeCall1() {
assertPrint("foo(a);", "foo(a)");
assertPrint("x.foo(a);", "x.foo(a)");
}
public void testFreeCall2() {
Node n = parse("foo(a);");
assertPrintNode("foo(a)", n);
Node call = n.getFirstChild().getFirstChild();
assertTrue(call.getType() == Token.CALL);
call.putBooleanProp(Node.FREE_CALL, true);
assertPrintNode("foo(a)", n);
}
public void testFreeCall3() {
Node n = parse("x.foo(a);");
assertPrintNode("x.foo(a)", n);
Node call = n.getFirstChild().getFirstChild();
assertTrue(call.getType() == Token.CALL);
call.putBooleanProp(Node.FREE_CALL, true);
assertPrintNode("(0,x.foo)(a)", n);
}
public void testPrintScript() {
// Verify that SCRIPT nodes not marked as synthetic are printed as
// blocks.
Node ast = new Node(Token.SCRIPT,
new Node(Token.EXPR_RESULT, Node.newString("f")),
new Node(Token.EXPR_RESULT, Node.newString("g")));
String result = new CodePrinter.Builder(ast).setPrettyPrint(true).build();
assertEquals("\"f\";\n\"g\";\n", result);
}
public void testObjectLit() {
assertPrint("({x:1})", "({x:1})");
assertPrint("var x=({x:1})", "var x={x:1}");
assertPrint("var x={'x':1}", "var x={\"x\":1}");
assertPrint("var x={1:1}", "var x={1:1}");
}
public void testObjectLit2() {
assertPrint("var x={1:1}", "var x={1:1}");
assertPrint("var x={'1':1}", "var x={1:1}");
assertPrint("var x={'1.0':1}", "var x={\"1.0\":1}");
assertPrint("var x={1.5:1}", "var x={\"1.5\":1}");
}
public void testObjectLit3() {
assertPrint("var x={3E9:1}",
"var x={3E9:1}");
assertPrint("var x={'3000000000':1}", // More than 31 bits
"var x={3E9:1}");
assertPrint("var x={'3000000001':1}",
"var x={3000000001:1}");
assertPrint("var x={'6000000001':1}", // More than 32 bits
"var x={6000000001:1}");
assertPrint("var x={\"12345678901234567\":1}", // More than 53 bits
"var x={\"12345678901234567\":1}");
}
public void testObjectLit4() {
// More than 128 bits.
assertPrint(
"var x={\"123456789012345671234567890123456712345678901234567\":1}",
"var x={\"123456789012345671234567890123456712345678901234567\":1}");
}
public void testGetter() {
assertPrint("var x = {}", "var x={}");
assertPrint("var x = {get a() {return 1}}", "var x={get a(){return 1}}");
assertPrint(
"var x = {get a() {}, get b(){}}",
"var x={get a(){},get b(){}}");
assertPrint(
"var x = {get 'a'() {return 1}}",
"var x={get \"a\"(){return 1}}");
assertPrint(
"var x = {get 1() {return 1}}",
"var x={get 1(){return 1}}");
assertPrint(
"var x = {get \"()\"() {return 1}}",
"var x={get \"()\"(){return 1}}");
}
public void testSetter() {
assertPrint("var x = {}", "var x={}");
assertPrint(
"var x = {set a(y) {return 1}}",
"var x={set a(y){return 1}}");
assertPrint(
"var x = {get 'a'() {return 1}}",
"var x={get \"a\"(){return 1}}");
assertPrint(
"var x = {set 1(y) {return 1}}",
"var x={set 1(y){return 1}}");
assertPrint(
"var x = {set \"(x)\"(y) {return 1}}",
"var x={set \"(x)\"(y){return 1}}");
}
public void testNegCollapse() {
// Collapse the negative symbol on numbers at generation time,
// to match the Rhino behavior.
assertPrint("var x = - - 2;", "var x=2");
assertPrint("var x = - (2);", "var x=-2");
}
public void testStrict() {
String result = parsePrint("var x", false, false, 0, false, true);
assertEquals("'use strict';var x", result);
}
public void testArrayLiteral() {
assertPrint("var x = [,];","var x=[,]");
assertPrint("var x = [,,];","var x=[,,]");
assertPrint("var x = [,s,,];","var x=[,s,,]");
assertPrint("var x = [,s];","var x=[,s]");
assertPrint("var x = [s,];","var x=[s]");
}
public void testZero() {
assertPrint("var x ='\\0';", "var x=\"\\x00\"");
assertPrint("var x ='\\x00';", "var x=\"\\x00\"");
assertPrint("var x ='\\u0000';", "var x=\"\\x00\"");
assertPrint("var x ='\\u00003';", "var x=\"\\x003\"");
}
public void testUnicode() {
assertPrint("var x ='\\x0f';", "var x=\"\\u000f\"");
assertPrint("var x ='\\x68';", "var x=\"h\"");
assertPrint("var x ='\\x7f';", "var x=\"\\u007f\"");
}
public void testUnicodeKeyword() {
// keyword "if"
assertPrint("var \\u0069\\u0066 = 1;", "var i\\u0066=1");
// keyword "var"
assertPrint("var v\\u0061\\u0072 = 1;", "var va\\u0072=1");
// all are keyword "while"
assertPrint("var w\\u0068\\u0069\\u006C\\u0065 = 1;"
+ "\\u0077\\u0068il\\u0065 = 2;"
+ "\\u0077h\\u0069le = 3;",
"var whil\\u0065=1;whil\\u0065=2;whil\\u0065=3");
}
public void testNumericKeys() {
assertPrint("var x = {010: 1};", "var x={8:1}");
assertPrint("var x = {'010': 1};", "var x={\"010\":1}");
assertPrint("var x = {0x10: 1};", "var x={16:1}");
assertPrint("var x = {'0x10': 1};", "var x={\"0x10\":1}");
// I was surprised at this result too.
assertPrint("var x = {.2: 1};", "var x={\"0.2\":1}");
assertPrint("var x = {'.2': 1};", "var x={\".2\":1}");
assertPrint("var x = {0.2: 1};", "var x={\"0.2\":1}");
assertPrint("var x = {'0.2': 1};", "var x={\"0.2\":1}");
}
} | // You are a professional Java test case writer, please create a test case named `testNumericKeys` for the issue `Closure-569`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-569
//
// ## Issue-Title:
// Converts string properties into numbers in literal object definitions
//
// ## Issue-Description:
// **What steps will reproduce the problem?**
// 1. Minimize the following script:
//
// var lit = {"0102":"Zero One Zero Two"};
// alert(lit["0102"]);
//
// **What is the expected output? What do you see instead?**
//
// Expected:
// var lit={"0102":"Zero One Zero Two"};alert(lit["0102"]);
//
// Actual:
// var lit={102:"Zero One Zero Two"};alert(lit["0102"]);
//
// **What version of the product are you using? On what operating system?**
//
// r1459
//
// **Please provide any additional information below.**
//
//
public void testNumericKeys() {
| 1,269 | 52 | 1,256 | test/com/google/javascript/jscomp/CodePrinterTest.java | test | ```markdown
## Issue-ID: Closure-569
## Issue-Title:
Converts string properties into numbers in literal object definitions
## Issue-Description:
**What steps will reproduce the problem?**
1. Minimize the following script:
var lit = {"0102":"Zero One Zero Two"};
alert(lit["0102"]);
**What is the expected output? What do you see instead?**
Expected:
var lit={"0102":"Zero One Zero Two"};alert(lit["0102"]);
Actual:
var lit={102:"Zero One Zero Two"};alert(lit["0102"]);
**What version of the product are you using? On what operating system?**
r1459
**Please provide any additional information below.**
```
You are a professional Java test case writer, please create a test case named `testNumericKeys` for the issue `Closure-569`, utilizing the provided issue report information and the following function signature.
```java
public void testNumericKeys() {
```
| 1,256 | [
"com.google.javascript.jscomp.CodeGenerator"
] | 8e5a080f1e0dbd57e1ca0d243745942665842d1bee60e0b4fbe377e3ecad9a6e | public void testNumericKeys() | // You are a professional Java test case writer, please create a test case named `testNumericKeys` for the issue `Closure-569`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-569
//
// ## Issue-Title:
// Converts string properties into numbers in literal object definitions
//
// ## Issue-Description:
// **What steps will reproduce the problem?**
// 1. Minimize the following script:
//
// var lit = {"0102":"Zero One Zero Two"};
// alert(lit["0102"]);
//
// **What is the expected output? What do you see instead?**
//
// Expected:
// var lit={"0102":"Zero One Zero Two"};alert(lit["0102"]);
//
// Actual:
// var lit={102:"Zero One Zero Two"};alert(lit["0102"]);
//
// **What version of the product are you using? On what operating system?**
//
// r1459
//
// **Please provide any additional information below.**
//
//
| Closure | /*
* Copyright 2004 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import com.google.javascript.jscomp.CompilerOptions.LanguageMode;
import com.google.javascript.rhino.InputId;
import com.google.javascript.rhino.Node;
import com.google.javascript.rhino.Token;
import junit.framework.TestCase;
public class CodePrinterTest extends TestCase {
static Node parse(String js) {
return parse(js, false);
}
static Node parse(String js, boolean checkTypes) {
Compiler compiler = new Compiler();
CompilerOptions options = new CompilerOptions();
// Allow getters and setters.
options.setLanguageIn(LanguageMode.ECMASCRIPT5);
compiler.initOptions(options);
Node n = compiler.parseTestCode(js);
if (checkTypes) {
DefaultPassConfig passConfig = new DefaultPassConfig(null);
CompilerPass typeResolver = passConfig.resolveTypes.create(compiler);
Node externs = new Node(Token.SCRIPT);
externs.setInputId(new InputId("externs"));
externs.setIsSyntheticBlock(true);
Node externAndJsRoot = new Node(Token.BLOCK, externs, n);
externAndJsRoot.setIsSyntheticBlock(true);
typeResolver.process(externs, n);
CompilerPass inferTypes = passConfig.inferTypes.create(compiler);
inferTypes.process(externs, n);
}
checkUnexpectedErrorsOrWarnings(compiler, 0);
return n;
}
private static void checkUnexpectedErrorsOrWarnings(
Compiler compiler, int expected) {
int actual = compiler.getErrors().length + compiler.getWarnings().length;
if (actual != expected) {
String msg = "";
for (JSError err : compiler.getErrors()) {
msg += "Error:" + err.toString() + "\n";
}
for (JSError err : compiler.getWarnings()) {
msg += "Warning:" + err.toString() + "\n";
}
assertEquals("Unexpected warnings or errors.\n " + msg, expected, actual);
}
}
String parsePrint(String js, boolean prettyprint, int lineThreshold) {
return new CodePrinter.Builder(parse(js)).setPrettyPrint(prettyprint)
.setLineLengthThreshold(lineThreshold).build();
}
String parsePrint(String js, boolean prettyprint, boolean lineBreak,
int lineThreshold) {
return new CodePrinter.Builder(parse(js)).setPrettyPrint(prettyprint)
.setLineLengthThreshold(lineThreshold).setLineBreak(lineBreak).build();
}
String parsePrint(String js, boolean prettyprint, boolean lineBreak,
int lineThreshold, boolean outputTypes) {
return new CodePrinter.Builder(parse(js, true)).setPrettyPrint(prettyprint)
.setOutputTypes(outputTypes)
.setLineLengthThreshold(lineThreshold).setLineBreak(lineBreak)
.build();
}
String parsePrint(String js, boolean prettyprint, boolean lineBreak,
int lineThreshold, boolean outputTypes,
boolean tagAsStrict) {
return new CodePrinter.Builder(parse(js, true)).setPrettyPrint(prettyprint)
.setOutputTypes(outputTypes)
.setLineLengthThreshold(lineThreshold).setLineBreak(lineBreak)
.setTagAsStrict(tagAsStrict)
.build();
}
String printNode(Node n) {
return new CodePrinter.Builder(n).setLineLengthThreshold(
CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD).build();
}
void assertPrintNode(String expectedJs, Node ast) {
assertEquals(expectedJs, printNode(ast));
}
public void testPrint() {
assertPrint("10 + a + b", "10+a+b");
assertPrint("10 + (30*50)", "10+30*50");
assertPrint("with(x) { x + 3; }", "with(x)x+3");
assertPrint("\"aa'a\"", "\"aa'a\"");
assertPrint("\"aa\\\"a\"", "'aa\"a'");
assertPrint("function foo()\n{return 10;}", "function foo(){return 10}");
assertPrint("a instanceof b", "a instanceof b");
assertPrint("typeof(a)", "typeof a");
assertPrint(
"var foo = x ? { a : 1 } : {a: 3, b:4, \"default\": 5, \"foo-bar\": 6}",
"var foo=x?{a:1}:{a:3,b:4,\"default\":5,\"foo-bar\":6}");
// Safari: needs ';' at the end of a throw statement
assertPrint("function foo(){throw 'error';}",
"function foo(){throw\"error\";}");
// Safari 3 needs a "{" around a single function
assertPrint("if (true) function foo(){return}",
"if(true){function foo(){return}}");
assertPrint("var x = 10; { var y = 20; }", "var x=10;var y=20");
assertPrint("while (x-- > 0);", "while(x-- >0);");
assertPrint("x-- >> 1", "x-- >>1");
assertPrint("(function () {})(); ",
"(function(){})()");
// Associativity
assertPrint("var a,b,c,d;a || (b&& c) && (a || d)",
"var a,b,c,d;a||b&&c&&(a||d)");
assertPrint("var a,b,c; a || (b || c); a * (b * c); a | (b | c)",
"var a,b,c;a||b||c;a*b*c;a|b|c");
assertPrint("var a,b,c; a / b / c;a / (b / c); a - (b - c);",
"var a,b,c;a/b/c;a/(b/c);a-(b-c)");
assertPrint("var a,b; a = b = 3;",
"var a,b;a=b=3");
assertPrint("var a,b,c,d; a = (b = c = (d = 3));",
"var a,b,c,d;a=b=c=d=3");
assertPrint("var a,b,c; a += (b = c += 3);",
"var a,b,c;a+=b=c+=3");
assertPrint("var a,b,c; a *= (b -= c);",
"var a,b,c;a*=b-=c");
// Break scripts
assertPrint("'<script>'", "\"<script>\"");
assertPrint("'</script>'", "\"<\\/script>\"");
assertPrint("\"</script> </SCRIPT>\"", "\"<\\/script> <\\/SCRIPT>\"");
assertPrint("'-->'", "\"--\\>\"");
assertPrint("']]>'", "\"]]\\>\"");
assertPrint("' --></script>'", "\" --\\><\\/script>\"");
assertPrint("/--> <\\/script>/g", "/--\\> <\\/script>/g");
// Break HTML start comments. Certain versions of Webkit
// begin an HTML comment when they see this.
assertPrint("'<!-- I am a string -->'", "\"<\\!-- I am a string --\\>\"");
// Precedence
assertPrint("a ? delete b[0] : 3", "a?delete b[0]:3");
assertPrint("(delete a[0])/10", "delete a[0]/10");
// optional '()' for new
// simple new
assertPrint("new A", "new A");
assertPrint("new A()", "new A");
assertPrint("new A('x')", "new A(\"x\")");
// calling instance method directly after new
assertPrint("new A().a()", "(new A).a()");
assertPrint("(new A).a()", "(new A).a()");
// this case should be fixed
assertPrint("new A('y').a()", "(new A(\"y\")).a()");
// internal class
assertPrint("new A.B", "new A.B");
assertPrint("new A.B()", "new A.B");
assertPrint("new A.B('z')", "new A.B(\"z\")");
// calling instance method directly after new internal class
assertPrint("(new A.B).a()", "(new A.B).a()");
assertPrint("new A.B().a()", "(new A.B).a()");
// this case should be fixed
assertPrint("new A.B('w').a()", "(new A.B(\"w\")).a()");
// Operators: make sure we don't convert binary + and unary + into ++
assertPrint("x + +y", "x+ +y");
assertPrint("x - (-y)", "x- -y");
assertPrint("x++ +y", "x++ +y");
assertPrint("x-- -y", "x-- -y");
assertPrint("x++ -y", "x++-y");
// Label
assertPrint("foo:for(;;){break foo;}", "foo:for(;;)break foo");
assertPrint("foo:while(1){continue foo;}", "foo:while(1)continue foo");
// Object literals.
assertPrint("({})", "({})");
assertPrint("var x = {};", "var x={}");
assertPrint("({}).x", "({}).x");
assertPrint("({})['x']", "({})[\"x\"]");
assertPrint("({}) instanceof Object", "({})instanceof Object");
assertPrint("({}) || 1", "({})||1");
assertPrint("1 || ({})", "1||{}");
assertPrint("({}) ? 1 : 2", "({})?1:2");
assertPrint("0 ? ({}) : 2", "0?{}:2");
assertPrint("0 ? 1 : ({})", "0?1:{}");
assertPrint("typeof ({})", "typeof{}");
assertPrint("f({})", "f({})");
// Anonymous function expressions.
assertPrint("(function(){})", "(function(){})");
assertPrint("(function(){})()", "(function(){})()");
assertPrint("(function(){})instanceof Object",
"(function(){})instanceof Object");
assertPrint("(function(){}).bind().call()",
"(function(){}).bind().call()");
assertPrint("var x = function() { };", "var x=function(){}");
assertPrint("var x = function() { }();", "var x=function(){}()");
assertPrint("(function() {}), 2", "(function(){}),2");
// Name functions expression.
assertPrint("(function f(){})", "(function f(){})");
// Function declaration.
assertPrint("function f(){}", "function f(){}");
// Make sure we don't treat non-latin character escapes as raw strings.
assertPrint("({ 'a': 4, '\\u0100': 4 })", "({\"a\":4,\"\\u0100\":4})");
assertPrint("({ a: 4, '\\u0100': 4 })", "({a:4,\"\\u0100\":4})");
// Test if statement and for statements with single statements in body.
assertPrint("if (true) { alert();}", "if(true)alert()");
assertPrint("if (false) {} else {alert(\"a\");}",
"if(false);else alert(\"a\")");
assertPrint("for(;;) { alert();};", "for(;;)alert()");
assertPrint("do { alert(); } while(true);",
"do alert();while(true)");
assertPrint("myLabel: { alert();}",
"myLabel:alert()");
assertPrint("myLabel: for(;;) continue myLabel;",
"myLabel:for(;;)continue myLabel");
// Test nested var statement
assertPrint("if (true) var x; x = 4;", "if(true)var x;x=4");
// Non-latin identifier. Make sure we keep them escaped.
assertPrint("\\u00fb", "\\u00fb");
assertPrint("\\u00fa=1", "\\u00fa=1");
assertPrint("function \\u00f9(){}", "function \\u00f9(){}");
assertPrint("x.\\u00f8", "x.\\u00f8");
assertPrint("x.\\u00f8", "x.\\u00f8");
assertPrint("abc\\u4e00\\u4e01jkl", "abc\\u4e00\\u4e01jkl");
// Test the right-associative unary operators for spurious parens
assertPrint("! ! true", "!!true");
assertPrint("!(!(true))", "!!true");
assertPrint("typeof(void(0))", "typeof void 0");
assertPrint("typeof(void(!0))", "typeof void!0");
assertPrint("+ - + + - + 3", "+-+ +-+3"); // chained unary plus/minus
assertPrint("+(--x)", "+--x");
assertPrint("-(++x)", "-++x");
// needs a space to prevent an ambiguous parse
assertPrint("-(--x)", "- --x");
assertPrint("!(~~5)", "!~~5");
assertPrint("~(a/b)", "~(a/b)");
// Preserve parens to overcome greedy binding of NEW
assertPrint("new (foo.bar()).factory(baz)", "new (foo.bar().factory)(baz)");
assertPrint("new (bar()).factory(baz)", "new (bar().factory)(baz)");
assertPrint("new (new foobar(x)).factory(baz)",
"new (new foobar(x)).factory(baz)");
// Make sure that HOOK is right associative
assertPrint("a ? b : (c ? d : e)", "a?b:c?d:e");
assertPrint("a ? (b ? c : d) : e", "a?b?c:d:e");
assertPrint("(a ? b : c) ? d : e", "(a?b:c)?d:e");
// Test nested ifs
assertPrint("if (x) if (y); else;", "if(x)if(y);else;");
// Test comma.
assertPrint("a,b,c", "a,b,c");
assertPrint("(a,b),c", "a,b,c");
assertPrint("a,(b,c)", "a,b,c");
assertPrint("x=a,b,c", "x=a,b,c");
assertPrint("x=(a,b),c", "x=(a,b),c");
assertPrint("x=a,(b,c)", "x=a,b,c");
assertPrint("x=a,y=b,z=c", "x=a,y=b,z=c");
assertPrint("x=(a,y=b,z=c)", "x=(a,y=b,z=c)");
assertPrint("x=[a,b,c,d]", "x=[a,b,c,d]");
assertPrint("x=[(a,b,c),d]", "x=[(a,b,c),d]");
assertPrint("x=[(a,(b,c)),d]", "x=[(a,b,c),d]");
assertPrint("x=[a,(b,c,d)]", "x=[a,(b,c,d)]");
assertPrint("var x=(a,b)", "var x=(a,b)");
assertPrint("var x=a,b,c", "var x=a,b,c");
assertPrint("var x=(a,b),c", "var x=(a,b),c");
assertPrint("var x=a,b=(c,d)", "var x=a,b=(c,d)");
assertPrint("foo(a,b,c,d)", "foo(a,b,c,d)");
assertPrint("foo((a,b,c),d)", "foo((a,b,c),d)");
assertPrint("foo((a,(b,c)),d)", "foo((a,b,c),d)");
assertPrint("f(a+b,(c,d,(e,f,g)))", "f(a+b,(c,d,e,f,g))");
assertPrint("({}) , 1 , 2", "({}),1,2");
assertPrint("({}) , {} , {}", "({}),{},{}");
// EMPTY nodes
assertPrint("if (x){}", "if(x);");
assertPrint("if(x);", "if(x);");
assertPrint("if(x)if(y);", "if(x)if(y);");
assertPrint("if(x){if(y);}", "if(x)if(y);");
assertPrint("if(x){if(y){};;;}", "if(x)if(y);");
assertPrint("if(x){;;function y(){};;}", "if(x){function y(){}}");
}
public void testPrintArray() {
assertPrint("[void 0, void 0]", "[void 0,void 0]");
assertPrint("[undefined, undefined]", "[undefined,undefined]");
assertPrint("[ , , , undefined]", "[,,,undefined]");
assertPrint("[ , , , 0]", "[,,,0]");
}
public void testHook() {
assertPrint("a ? b = 1 : c = 2", "a?b=1:c=2");
assertPrint("x = a ? b = 1 : c = 2", "x=a?b=1:c=2");
assertPrint("(x = a) ? b = 1 : c = 2", "(x=a)?b=1:c=2");
assertPrint("x, a ? b = 1 : c = 2", "x,a?b=1:c=2");
assertPrint("x, (a ? b = 1 : c = 2)", "x,a?b=1:c=2");
assertPrint("(x, a) ? b = 1 : c = 2", "(x,a)?b=1:c=2");
assertPrint("a ? (x, b) : c = 2", "a?(x,b):c=2");
assertPrint("a ? b = 1 : (x,c)", "a?b=1:(x,c)");
assertPrint("a ? b = 1 : c = 2 + x", "a?b=1:c=2+x");
assertPrint("(a ? b = 1 : c = 2) + x", "(a?b=1:c=2)+x");
assertPrint("a ? b = 1 : (c = 2) + x", "a?b=1:(c=2)+x");
assertPrint("a ? (b?1:2) : 3", "a?b?1:2:3");
}
public void testPrintInOperatorInForLoop() {
// Check for in expression in for's init expression.
// Check alone, with + (higher precedence), with ?: (lower precedence),
// and with conditional.
assertPrint("var a={}; for (var i = (\"length\" in a); i;) {}",
"var a={};for(var i=(\"length\"in a);i;);");
assertPrint("var a={}; for (var i = (\"length\" in a) ? 0 : 1; i;) {}",
"var a={};for(var i=(\"length\"in a)?0:1;i;);");
assertPrint("var a={}; for (var i = (\"length\" in a) + 1; i;) {}",
"var a={};for(var i=(\"length\"in a)+1;i;);");
assertPrint("var a={};for (var i = (\"length\" in a|| \"size\" in a);;);",
"var a={};for(var i=(\"length\"in a)||(\"size\"in a);;);");
assertPrint("var a={};for (var i = a || a || (\"size\" in a);;);",
"var a={};for(var i=a||a||(\"size\"in a);;);");
// Test works with unary operators and calls.
assertPrint("var a={}; for (var i = -(\"length\" in a); i;) {}",
"var a={};for(var i=-(\"length\"in a);i;);");
assertPrint("var a={};function b_(p){ return p;};" +
"for(var i=1,j=b_(\"length\" in a);;) {}",
"var a={};function b_(p){return p}" +
"for(var i=1,j=b_(\"length\"in a);;);");
// Test we correctly handle an in operator in the test clause.
assertPrint("var a={}; for (;(\"length\" in a);) {}",
"var a={};for(;\"length\"in a;);");
}
public void testLiteralProperty() {
assertPrint("(64).toString()", "(64).toString()");
}
private void assertPrint(String js, String expected) {
parse(expected); // validate the expected string is valid js
assertEquals(expected,
parsePrint(js, false, CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD));
}
// Make sure that the code generator doesn't associate an
// else clause with the wrong if clause.
public void testAmbiguousElseClauses() {
assertPrintNode("if(x)if(y);else;",
new Node(Token.IF,
Node.newString(Token.NAME, "x"),
new Node(Token.BLOCK,
new Node(Token.IF,
Node.newString(Token.NAME, "y"),
new Node(Token.BLOCK),
// ELSE clause for the inner if
new Node(Token.BLOCK)))));
assertPrintNode("if(x){if(y);}else;",
new Node(Token.IF,
Node.newString(Token.NAME, "x"),
new Node(Token.BLOCK,
new Node(Token.IF,
Node.newString(Token.NAME, "y"),
new Node(Token.BLOCK))),
// ELSE clause for the outer if
new Node(Token.BLOCK)));
assertPrintNode("if(x)if(y);else{if(z);}else;",
new Node(Token.IF,
Node.newString(Token.NAME, "x"),
new Node(Token.BLOCK,
new Node(Token.IF,
Node.newString(Token.NAME, "y"),
new Node(Token.BLOCK),
new Node(Token.BLOCK,
new Node(Token.IF,
Node.newString(Token.NAME, "z"),
new Node(Token.BLOCK))))),
// ELSE clause for the outermost if
new Node(Token.BLOCK)));
}
public void testLineBreak() {
// line break after function if in a statement context
assertLineBreak("function a() {}\n" +
"function b() {}",
"function a(){}\n" +
"function b(){}\n");
// line break after ; after a function
assertLineBreak("var a = {};\n" +
"a.foo = function () {}\n" +
"function b() {}",
"var a={};a.foo=function(){};\n" +
"function b(){}\n");
// break after comma after a function
assertLineBreak("var a = {\n" +
" b: function() {},\n" +
" c: function() {}\n" +
"};\n" +
"alert(a);",
"var a={b:function(){},\n" +
"c:function(){}};\n" +
"alert(a)");
}
private void assertLineBreak(String js, String expected) {
assertEquals(expected,
parsePrint(js, false, true,
CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD));
}
public void testPrettyPrinter() {
// Ensure that the pretty printer inserts line breaks at appropriate
// places.
assertPrettyPrint("(function(){})();","(function() {\n})();\n");
assertPrettyPrint("var a = (function() {});alert(a);",
"var a = function() {\n};\nalert(a);\n");
// Check we correctly handle putting brackets around all if clauses so
// we can put breakpoints inside statements.
assertPrettyPrint("if (1) {}",
"if(1) {\n" +
"}\n");
assertPrettyPrint("if (1) {alert(\"\");}",
"if(1) {\n" +
" alert(\"\")\n" +
"}\n");
assertPrettyPrint("if (1)alert(\"\");",
"if(1) {\n" +
" alert(\"\")\n" +
"}\n");
assertPrettyPrint("if (1) {alert();alert();}",
"if(1) {\n" +
" alert();\n" +
" alert()\n" +
"}\n");
// Don't add blocks if they weren't there already.
assertPrettyPrint("label: alert();",
"label:alert();\n");
// But if statements and loops get blocks automagically.
assertPrettyPrint("if (1) alert();",
"if(1) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint("for (;;) alert();",
"for(;;) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint("while (1) alert();",
"while(1) {\n" +
" alert()\n" +
"}\n");
// Do we put else clauses in blocks?
assertPrettyPrint("if (1) {} else {alert(a);}",
"if(1) {\n" +
"}else {\n alert(a)\n}\n");
// Do we add blocks to else clauses?
assertPrettyPrint("if (1) alert(a); else alert(b);",
"if(1) {\n" +
" alert(a)\n" +
"}else {\n" +
" alert(b)\n" +
"}\n");
// Do we put for bodies in blocks?
assertPrettyPrint("for(;;) { alert();}",
"for(;;) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint("for(;;) {}",
"for(;;) {\n" +
"}\n");
assertPrettyPrint("for(;;) { alert(); alert(); }",
"for(;;) {\n" +
" alert();\n" +
" alert()\n" +
"}\n");
// How about do loops?
assertPrettyPrint("do { alert(); } while(true);",
"do {\n" +
" alert()\n" +
"}while(true);\n");
// label?
assertPrettyPrint("myLabel: { alert();}",
"myLabel: {\n" +
" alert()\n" +
"}\n");
// Don't move the label on a loop, because then break {label} and
// continue {label} won't work.
assertPrettyPrint("myLabel: for(;;) continue myLabel;",
"myLabel:for(;;) {\n" +
" continue myLabel\n" +
"}\n");
assertPrettyPrint("var a;", "var a;\n");
}
public void testPrettyPrinter2() {
assertPrettyPrint(
"if(true) f();",
"if(true) {\n" +
" f()\n" +
"}\n");
assertPrettyPrint(
"if (true) { f() } else { g() }",
"if(true) {\n" +
" f()\n" +
"}else {\n" +
" g()\n" +
"}\n");
assertPrettyPrint(
"if(true) f(); for(;;) g();",
"if(true) {\n" +
" f()\n" +
"}\n" +
"for(;;) {\n" +
" g()\n" +
"}\n");
}
public void testPrettyPrinter3() {
assertPrettyPrint(
"try {} catch(e) {}if (1) {alert();alert();}",
"try {\n" +
"}catch(e) {\n" +
"}\n" +
"if(1) {\n" +
" alert();\n" +
" alert()\n" +
"}\n");
assertPrettyPrint(
"try {} finally {}if (1) {alert();alert();}",
"try {\n" +
"}finally {\n" +
"}\n" +
"if(1) {\n" +
" alert();\n" +
" alert()\n" +
"}\n");
assertPrettyPrint(
"try {} catch(e) {} finally {} if (1) {alert();alert();}",
"try {\n" +
"}catch(e) {\n" +
"}finally {\n" +
"}\n" +
"if(1) {\n" +
" alert();\n" +
" alert()\n" +
"}\n");
}
public void testPrettyPrinter4() {
assertPrettyPrint(
"function f() {}if (1) {alert();}",
"function f() {\n" +
"}\n" +
"if(1) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint(
"var f = function() {};if (1) {alert();}",
"var f = function() {\n" +
"};\n" +
"if(1) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint(
"(function() {})();if (1) {alert();}",
"(function() {\n" +
"})();\n" +
"if(1) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint(
"(function() {alert();alert();})();if (1) {alert();}",
"(function() {\n" +
" alert();\n" +
" alert()\n" +
"})();\n" +
"if(1) {\n" +
" alert()\n" +
"}\n");
}
public void testTypeAnnotations() {
assertTypeAnnotations(
"/** @constructor */ function Foo(){}",
"/**\n * @return {undefined}\n * @constructor\n */\n"
+ "function Foo() {\n}\n");
}
public void testTypeAnnotationsTypeDef() {
// TODO(johnlenz): It would be nice if there were some way to preserve
// typedefs but currently they are resolved into the basic types in the
// type registry.
assertTypeAnnotations(
"/** @typedef {Array.<number>} */ goog.java.Long;\n"
+ "/** @param {!goog.java.Long} a*/\n"
+ "function f(a){};\n",
"goog.java.Long;\n"
+ "/**\n"
+ " * @param {(Array|null)} a\n"
+ " * @return {undefined}\n"
+ " */\n"
+ "function f(a) {\n}\n");
}
public void testTypeAnnotationsAssign() {
assertTypeAnnotations("/** @constructor */ var Foo = function(){}",
"/**\n * @return {undefined}\n * @constructor\n */\n"
+ "var Foo = function() {\n};\n");
}
public void testTypeAnnotationsNamespace() {
assertTypeAnnotations("var a = {};"
+ "/** @constructor */ a.Foo = function(){}",
"var a = {};\n"
+ "/**\n * @return {undefined}\n * @constructor\n */\n"
+ "a.Foo = function() {\n};\n");
}
public void testTypeAnnotationsMemberSubclass() {
assertTypeAnnotations("var a = {};"
+ "/** @constructor */ a.Foo = function(){};"
+ "/** @constructor \n @extends {a.Foo} */ a.Bar = function(){}",
"var a = {};\n"
+ "/**\n * @return {undefined}\n * @constructor\n */\n"
+ "a.Foo = function() {\n};\n"
+ "/**\n * @return {undefined}\n * @extends {a.Foo}\n"
+ " * @constructor\n */\n"
+ "a.Bar = function() {\n};\n");
}
public void testTypeAnnotationsInterface() {
assertTypeAnnotations("var a = {};"
+ "/** @interface */ a.Foo = function(){};"
+ "/** @interface \n @extends {a.Foo} */ a.Bar = function(){}",
"var a = {};\n"
+ "/**\n * @interface\n */\n"
+ "a.Foo = function() {\n};\n"
+ "/**\n * @extends {a.Foo}\n"
+ " * @interface\n */\n"
+ "a.Bar = function() {\n};\n");
}
public void testTypeAnnotationsMultipleInterface() {
assertTypeAnnotations("var a = {};"
+ "/** @interface */ a.Foo1 = function(){};"
+ "/** @interface */ a.Foo2 = function(){};"
+ "/** @interface \n @extends {a.Foo1} \n @extends {a.Foo2} */"
+ "a.Bar = function(){}",
"var a = {};\n"
+ "/**\n * @interface\n */\n"
+ "a.Foo1 = function() {\n};\n"
+ "/**\n * @interface\n */\n"
+ "a.Foo2 = function() {\n};\n"
+ "/**\n * @extends {a.Foo1}\n"
+ " * @extends {a.Foo2}\n"
+ " * @interface\n */\n"
+ "a.Bar = function() {\n};\n");
}
public void testTypeAnnotationsMember() {
assertTypeAnnotations("var a = {};"
+ "/** @constructor */ a.Foo = function(){}"
+ "/** @param {string} foo\n"
+ " * @return {number} */\n"
+ "a.Foo.prototype.foo = function(foo) { return 3; };"
+ "/** @type {string|undefined} */"
+ "a.Foo.prototype.bar = '';",
"var a = {};\n"
+ "/**\n * @return {undefined}\n * @constructor\n */\n"
+ "a.Foo = function() {\n};\n"
+ "/**\n"
+ " * @param {string} foo\n"
+ " * @return {number}\n"
+ " */\n"
+ "a.Foo.prototype.foo = function(foo) {\n return 3\n};\n"
+ "/** @type {string} */\n"
+ "a.Foo.prototype.bar = \"\";\n");
}
public void testTypeAnnotationsImplements() {
assertTypeAnnotations("var a = {};"
+ "/** @constructor */ a.Foo = function(){};\n"
+ "/** @interface */ a.I = function(){};\n"
+ "/** @interface */ a.I2 = function(){};\n"
+ "/** @constructor \n @extends {a.Foo}\n"
+ " * @implements {a.I} \n @implements {a.I2}\n"
+ "*/ a.Bar = function(){}",
"var a = {};\n"
+ "/**\n * @return {undefined}\n * @constructor\n */\n"
+ "a.Foo = function() {\n};\n"
+ "/**\n * @interface\n */\n"
+ "a.I = function() {\n};\n"
+ "/**\n * @interface\n */\n"
+ "a.I2 = function() {\n};\n"
+ "/**\n * @return {undefined}\n * @extends {a.Foo}\n"
+ " * @implements {a.I}\n"
+ " * @implements {a.I2}\n * @constructor\n */\n"
+ "a.Bar = function() {\n};\n");
}
public void testTypeAnnotationsDispatcher1() {
assertTypeAnnotations(
"var a = {};\n" +
"/** \n" +
" * @constructor \n" +
" * @javadispatch \n" +
" */\n" +
"a.Foo = function(){}",
"var a = {};\n" +
"/**\n" +
" * @return {undefined}\n" +
" * @constructor\n" +
" * @javadispatch\n" +
" */\n" +
"a.Foo = function() {\n" +
"};\n");
}
public void testTypeAnnotationsDispatcher2() {
assertTypeAnnotations(
"var a = {};\n" +
"/** \n" +
" * @constructor \n" +
" */\n" +
"a.Foo = function(){}\n" +
"/**\n" +
" * @javadispatch\n" +
" */\n" +
"a.Foo.prototype.foo = function() {};",
"var a = {};\n" +
"/**\n" +
" * @return {undefined}\n" +
" * @constructor\n" +
" */\n" +
"a.Foo = function() {\n" +
"};\n" +
"/**\n" +
" * @return {undefined}\n" +
" * @javadispatch\n" +
" */\n" +
"a.Foo.prototype.foo = function() {\n" +
"};\n");
}
public void testU2UFunctionTypeAnnotation() {
assertTypeAnnotations(
"/** @type {!Function} */ var x = function() {}",
"/**\n * @constructor\n */\nvar x = function() {\n};\n");
}
public void testEmitUnknownParamTypesAsAllType() {
assertTypeAnnotations(
"var a = function(x) {}",
"/**\n" +
" * @param {*} x\n" +
" * @return {undefined}\n" +
" */\n" +
"var a = function(x) {\n};\n");
}
public void testOptionalTypesAnnotation() {
assertTypeAnnotations(
"/**\n" +
" * @param {string=} x \n" +
" */\n" +
"var a = function(x) {}",
"/**\n" +
" * @param {string=} x\n" +
" * @return {undefined}\n" +
" */\n" +
"var a = function(x) {\n};\n");
}
public void testVariableArgumentsTypesAnnotation() {
assertTypeAnnotations(
"/**\n" +
" * @param {...string} x \n" +
" */\n" +
"var a = function(x) {}",
"/**\n" +
" * @param {...string} x\n" +
" * @return {undefined}\n" +
" */\n" +
"var a = function(x) {\n};\n");
}
public void testTempConstructor() {
assertTypeAnnotations(
"var x = function() {\n/**\n * @constructor\n */\nfunction t1() {}\n" +
" /**\n * @constructor\n */\nfunction t2() {}\n" +
" t1.prototype = t2.prototype}",
"/**\n * @return {undefined}\n */\nvar x = function() {\n" +
" /**\n * @return {undefined}\n * @constructor\n */\n" +
"function t1() {\n }\n" +
" /**\n * @return {undefined}\n * @constructor\n */\n" +
"function t2() {\n }\n" +
" t1.prototype = t2.prototype\n};\n"
);
}
private void assertPrettyPrint(String js, String expected) {
assertEquals(expected,
parsePrint(js, true, false,
CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD));
}
private void assertTypeAnnotations(String js, String expected) {
assertEquals(expected,
parsePrint(js, true, false,
CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD, true));
}
public void testSubtraction() {
Compiler compiler = new Compiler();
Node n = compiler.parseTestCode("x - -4");
assertEquals(0, compiler.getErrorCount());
assertEquals(
"x- -4",
new CodePrinter.Builder(n).setLineLengthThreshold(
CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD).build());
}
public void testFunctionWithCall() {
assertPrint(
"var user = new function() {"
+ "alert(\"foo\")}",
"var user=new function(){"
+ "alert(\"foo\")}");
assertPrint(
"var user = new function() {"
+ "this.name = \"foo\";"
+ "this.local = function(){alert(this.name)};}",
"var user=new function(){"
+ "this.name=\"foo\";"
+ "this.local=function(){alert(this.name)}}");
}
public void testLineLength() {
// list
assertLineLength("var aba,bcb,cdc",
"var aba,bcb," +
"\ncdc");
// operators, and two breaks
assertLineLength(
"\"foo\"+\"bar,baz,bomb\"+\"whee\"+\";long-string\"\n+\"aaa\"",
"\"foo\"+\"bar,baz,bomb\"+" +
"\n\"whee\"+\";long-string\"+" +
"\n\"aaa\"");
// assignment
assertLineLength("var abazaba=1234",
"var abazaba=" +
"\n1234");
// statements
assertLineLength("var abab=1;var bab=2",
"var abab=1;" +
"\nvar bab=2");
// don't break regexes
assertLineLength("var a=/some[reg](ex),with.*we?rd|chars/i;var b=a",
"var a=/some[reg](ex),with.*we?rd|chars/i;" +
"\nvar b=a");
// don't break strings
assertLineLength("var a=\"foo,{bar};baz\";var b=a",
"var a=\"foo,{bar};baz\";" +
"\nvar b=a");
// don't break before post inc/dec
assertLineLength("var a=\"a\";a++;var b=\"bbb\";",
"var a=\"a\";a++;\n" +
"var b=\"bbb\"");
}
private void assertLineLength(String js, String expected) {
assertEquals(expected,
parsePrint(js, false, true, 10));
}
public void testParsePrintParse() {
testReparse("3;");
testReparse("var a = b;");
testReparse("var x, y, z;");
testReparse("try { foo() } catch(e) { bar() }");
testReparse("try { foo() } catch(e) { bar() } finally { stuff() }");
testReparse("try { foo() } finally { stuff() }");
testReparse("throw 'me'");
testReparse("function foo(a) { return a + 4; }");
testReparse("function foo() { return; }");
testReparse("var a = function(a, b) { foo(); return a + b; }");
testReparse("b = [3, 4, 'paul', \"Buchhe it\",,5];");
testReparse("v = (5, 6, 7, 8)");
testReparse("d = 34.0; x = 0; y = .3; z = -22");
testReparse("d = -x; t = !x + ~y;");
testReparse("'hi'; /* just a test */ stuff(a,b) \n" +
" foo(); // and another \n" +
" bar();");
testReparse("a = b++ + ++c; a = b++-++c; a = - --b; a = - ++b;");
testReparse("a++; b= a++; b = ++a; b = a--; b = --a; a+=2; b-=5");
testReparse("a = (2 + 3) * 4;");
testReparse("a = 1 + (2 + 3) + 4;");
testReparse("x = a ? b : c; x = a ? (b,3,5) : (foo(),bar());");
testReparse("a = b | c || d ^ e " +
"&& f & !g != h << i <= j < k >>> l > m * n % !o");
testReparse("a == b; a != b; a === b; a == b == a;" +
" (a == b) == a; a == (b == a);");
testReparse("if (a > b) a = b; if (b < 3) a = 3; else c = 4;");
testReparse("if (a == b) { a++; } if (a == 0) { a++; } else { a --; }");
testReparse("for (var i in a) b += i;");
testReparse("for (var i = 0; i < 10; i++){ b /= 2;" +
" if (b == 2)break;else continue;}");
testReparse("for (x = 0; x < 10; x++) a /= 2;");
testReparse("for (;;) a++;");
testReparse("while(true) { blah(); }while(true) blah();");
testReparse("do stuff(); while(a>b);");
testReparse("[0, null, , true, false, this];");
testReparse("s.replace(/absc/, 'X').replace(/ab/gi, 'Y');");
testReparse("new Foo; new Bar(a, b,c);");
testReparse("with(foo()) { x = z; y = t; } with(bar()) a = z;");
testReparse("delete foo['bar']; delete foo;");
testReparse("var x = { 'a':'paul', 1:'3', 2:(3,4) };");
testReparse("switch(a) { case 2: case 3: stuff(); break;" +
"case 4: morestuff(); break; default: done();}");
testReparse("x = foo['bar'] + foo['my stuff'] + foo[bar] + f.stuff;");
testReparse("a.v = b.v; x['foo'] = y['zoo'];");
testReparse("'test' in x; 3 in x; a in x;");
testReparse("'foo\"bar' + \"foo'c\" + 'stuff\\n and \\\\more'");
testReparse("x.__proto__;");
}
private void testReparse(String code) {
Compiler compiler = new Compiler();
Node parse1 = parse(code);
Node parse2 = parse(new CodePrinter.Builder(parse1).build());
String explanation = parse1.checkTreeEquals(parse2);
assertNull("\nExpected: " + compiler.toSource(parse1) +
"\nResult: " + compiler.toSource(parse2) +
"\n" + explanation, explanation);
}
public void testDoLoopIECompatiblity() {
// Do loops within IFs cause syntax errors in IE6 and IE7.
assertPrint("function f(){if(e1){do foo();while(e2)}else foo()}",
"function f(){if(e1){do foo();while(e2)}else foo()}");
assertPrint("function f(){if(e1)do foo();while(e2)else foo()}",
"function f(){if(e1){do foo();while(e2)}else foo()}");
assertPrint("if(x){do{foo()}while(y)}else bar()",
"if(x){do foo();while(y)}else bar()");
assertPrint("if(x)do{foo()}while(y);else bar()",
"if(x){do foo();while(y)}else bar()");
assertPrint("if(x){do{foo()}while(y)}",
"if(x){do foo();while(y)}");
assertPrint("if(x)do{foo()}while(y);",
"if(x){do foo();while(y)}");
assertPrint("if(x)A:do{foo()}while(y);",
"if(x){A:do foo();while(y)}");
assertPrint("var i = 0;a: do{b: do{i++;break b;} while(0);} while(0);",
"var i=0;a:do{b:do{i++;break b}while(0)}while(0)");
}
public void testFunctionSafariCompatiblity() {
// Functions within IFs cause syntax errors on Safari.
assertPrint("function f(){if(e1){function goo(){return true}}else foo()}",
"function f(){if(e1){function goo(){return true}}else foo()}");
assertPrint("function f(){if(e1)function goo(){return true}else foo()}",
"function f(){if(e1){function goo(){return true}}else foo()}");
assertPrint("if(e1){function goo(){return true}}",
"if(e1){function goo(){return true}}");
assertPrint("if(e1)function goo(){return true}",
"if(e1){function goo(){return true}}");
assertPrint("if(e1)A:function goo(){return true}",
"if(e1){A:function goo(){return true}}");
}
public void testExponents() {
assertPrintNumber("1", 1);
assertPrintNumber("10", 10);
assertPrintNumber("100", 100);
assertPrintNumber("1E3", 1000);
assertPrintNumber("1E4", 10000);
assertPrintNumber("1E5", 100000);
assertPrintNumber("-1", -1);
assertPrintNumber("-10", -10);
assertPrintNumber("-100", -100);
assertPrintNumber("-1E3", -1000);
assertPrintNumber("-12341234E4", -123412340000L);
assertPrintNumber("1E18", 1000000000000000000L);
assertPrintNumber("1E5", 100000.0);
assertPrintNumber("100000.1", 100000.1);
assertPrintNumber("1.0E-6", 0.000001);
}
// Make sure to test as both a String and a Node, because
// negative numbers do not parse consistently from strings.
private void assertPrintNumber(String expected, double number) {
assertPrint(String.valueOf(number), expected);
assertPrintNode(expected, Node.newNumber(number));
}
private void assertPrintNumber(String expected, int number) {
assertPrint(String.valueOf(number), expected);
assertPrintNode(expected, Node.newNumber(number));
}
public void testDirectEval() {
assertPrint("eval('1');", "eval(\"1\")");
}
public void testIndirectEval() {
Node n = parse("eval('1');");
assertPrintNode("eval(\"1\")", n);
n.getFirstChild().getFirstChild().getFirstChild().putBooleanProp(
Node.DIRECT_EVAL, false);
assertPrintNode("(0,eval)(\"1\")", n);
}
public void testFreeCall1() {
assertPrint("foo(a);", "foo(a)");
assertPrint("x.foo(a);", "x.foo(a)");
}
public void testFreeCall2() {
Node n = parse("foo(a);");
assertPrintNode("foo(a)", n);
Node call = n.getFirstChild().getFirstChild();
assertTrue(call.getType() == Token.CALL);
call.putBooleanProp(Node.FREE_CALL, true);
assertPrintNode("foo(a)", n);
}
public void testFreeCall3() {
Node n = parse("x.foo(a);");
assertPrintNode("x.foo(a)", n);
Node call = n.getFirstChild().getFirstChild();
assertTrue(call.getType() == Token.CALL);
call.putBooleanProp(Node.FREE_CALL, true);
assertPrintNode("(0,x.foo)(a)", n);
}
public void testPrintScript() {
// Verify that SCRIPT nodes not marked as synthetic are printed as
// blocks.
Node ast = new Node(Token.SCRIPT,
new Node(Token.EXPR_RESULT, Node.newString("f")),
new Node(Token.EXPR_RESULT, Node.newString("g")));
String result = new CodePrinter.Builder(ast).setPrettyPrint(true).build();
assertEquals("\"f\";\n\"g\";\n", result);
}
public void testObjectLit() {
assertPrint("({x:1})", "({x:1})");
assertPrint("var x=({x:1})", "var x={x:1}");
assertPrint("var x={'x':1}", "var x={\"x\":1}");
assertPrint("var x={1:1}", "var x={1:1}");
}
public void testObjectLit2() {
assertPrint("var x={1:1}", "var x={1:1}");
assertPrint("var x={'1':1}", "var x={1:1}");
assertPrint("var x={'1.0':1}", "var x={\"1.0\":1}");
assertPrint("var x={1.5:1}", "var x={\"1.5\":1}");
}
public void testObjectLit3() {
assertPrint("var x={3E9:1}",
"var x={3E9:1}");
assertPrint("var x={'3000000000':1}", // More than 31 bits
"var x={3E9:1}");
assertPrint("var x={'3000000001':1}",
"var x={3000000001:1}");
assertPrint("var x={'6000000001':1}", // More than 32 bits
"var x={6000000001:1}");
assertPrint("var x={\"12345678901234567\":1}", // More than 53 bits
"var x={\"12345678901234567\":1}");
}
public void testObjectLit4() {
// More than 128 bits.
assertPrint(
"var x={\"123456789012345671234567890123456712345678901234567\":1}",
"var x={\"123456789012345671234567890123456712345678901234567\":1}");
}
public void testGetter() {
assertPrint("var x = {}", "var x={}");
assertPrint("var x = {get a() {return 1}}", "var x={get a(){return 1}}");
assertPrint(
"var x = {get a() {}, get b(){}}",
"var x={get a(){},get b(){}}");
assertPrint(
"var x = {get 'a'() {return 1}}",
"var x={get \"a\"(){return 1}}");
assertPrint(
"var x = {get 1() {return 1}}",
"var x={get 1(){return 1}}");
assertPrint(
"var x = {get \"()\"() {return 1}}",
"var x={get \"()\"(){return 1}}");
}
public void testSetter() {
assertPrint("var x = {}", "var x={}");
assertPrint(
"var x = {set a(y) {return 1}}",
"var x={set a(y){return 1}}");
assertPrint(
"var x = {get 'a'() {return 1}}",
"var x={get \"a\"(){return 1}}");
assertPrint(
"var x = {set 1(y) {return 1}}",
"var x={set 1(y){return 1}}");
assertPrint(
"var x = {set \"(x)\"(y) {return 1}}",
"var x={set \"(x)\"(y){return 1}}");
}
public void testNegCollapse() {
// Collapse the negative symbol on numbers at generation time,
// to match the Rhino behavior.
assertPrint("var x = - - 2;", "var x=2");
assertPrint("var x = - (2);", "var x=-2");
}
public void testStrict() {
String result = parsePrint("var x", false, false, 0, false, true);
assertEquals("'use strict';var x", result);
}
public void testArrayLiteral() {
assertPrint("var x = [,];","var x=[,]");
assertPrint("var x = [,,];","var x=[,,]");
assertPrint("var x = [,s,,];","var x=[,s,,]");
assertPrint("var x = [,s];","var x=[,s]");
assertPrint("var x = [s,];","var x=[s]");
}
public void testZero() {
assertPrint("var x ='\\0';", "var x=\"\\x00\"");
assertPrint("var x ='\\x00';", "var x=\"\\x00\"");
assertPrint("var x ='\\u0000';", "var x=\"\\x00\"");
assertPrint("var x ='\\u00003';", "var x=\"\\x003\"");
}
public void testUnicode() {
assertPrint("var x ='\\x0f';", "var x=\"\\u000f\"");
assertPrint("var x ='\\x68';", "var x=\"h\"");
assertPrint("var x ='\\x7f';", "var x=\"\\u007f\"");
}
public void testUnicodeKeyword() {
// keyword "if"
assertPrint("var \\u0069\\u0066 = 1;", "var i\\u0066=1");
// keyword "var"
assertPrint("var v\\u0061\\u0072 = 1;", "var va\\u0072=1");
// all are keyword "while"
assertPrint("var w\\u0068\\u0069\\u006C\\u0065 = 1;"
+ "\\u0077\\u0068il\\u0065 = 2;"
+ "\\u0077h\\u0069le = 3;",
"var whil\\u0065=1;whil\\u0065=2;whil\\u0065=3");
}
public void testNumericKeys() {
assertPrint("var x = {010: 1};", "var x={8:1}");
assertPrint("var x = {'010': 1};", "var x={\"010\":1}");
assertPrint("var x = {0x10: 1};", "var x={16:1}");
assertPrint("var x = {'0x10': 1};", "var x={\"0x10\":1}");
// I was surprised at this result too.
assertPrint("var x = {.2: 1};", "var x={\"0.2\":1}");
assertPrint("var x = {'.2': 1};", "var x={\".2\":1}");
assertPrint("var x = {0.2: 1};", "var x={\"0.2\":1}");
assertPrint("var x = {'0.2': 1};", "var x={\"0.2\":1}");
}
} |
||
public void testDependencySorting() throws Exception {
CompilerOptions options = createCompilerOptions();
options.setDependencyOptions(
new DependencyOptions()
.setDependencySorting(true));
test(
options,
new String[] {
"goog.require('x');",
"goog.provide('x');",
},
new String[] {
"goog.provide('x');",
"goog.require('x');",
// For complicated reasons involving modules,
// the compiler creates a synthetic source file.
"",
});
} | com.google.javascript.jscomp.IntegrationTest::testDependencySorting | test/com/google/javascript/jscomp/IntegrationTest.java | 2,120 | test/com/google/javascript/jscomp/IntegrationTest.java | testDependencySorting | /*
* Copyright 2009 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import com.google.common.collect.ArrayListMultimap;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.Sets;
import com.google.javascript.jscomp.CompilerOptions.LanguageMode;
import com.google.javascript.rhino.Node;
import com.google.javascript.rhino.Token;
/**
* Tests for {@link PassFactory}.
*
* @author [email protected] (Nick Santos)
*/
public class IntegrationTest extends IntegrationTestCase {
private static final String CLOSURE_BOILERPLATE =
"/** @define {boolean} */ var COMPILED = false; var goog = {};" +
"goog.exportSymbol = function() {};";
private static final String CLOSURE_COMPILED =
"var COMPILED = true; var goog$exportSymbol = function() {};";
public void testBug1949424() {
CompilerOptions options = createCompilerOptions();
options.collapseProperties = true;
options.closurePass = true;
test(options, CLOSURE_BOILERPLATE + "goog.provide('FOO'); FOO.bar = 3;",
CLOSURE_COMPILED + "var FOO$bar = 3;");
}
public void testBug1949424_v2() {
CompilerOptions options = createCompilerOptions();
options.collapseProperties = true;
options.closurePass = true;
test(options, CLOSURE_BOILERPLATE + "goog.provide('FOO.BAR'); FOO.BAR = 3;",
CLOSURE_COMPILED + "var FOO$BAR = 3;");
}
public void testBug1956277() {
CompilerOptions options = createCompilerOptions();
options.collapseProperties = true;
options.inlineVariables = true;
test(options, "var CONST = {}; CONST.bar = null;" +
"function f(url) { CONST.bar = url; }",
"var CONST$bar = null; function f(url) { CONST$bar = url; }");
}
public void testBug1962380() {
CompilerOptions options = createCompilerOptions();
options.collapseProperties = true;
options.inlineVariables = true;
options.generateExports = true;
test(options,
CLOSURE_BOILERPLATE + "/** @export */ goog.CONSTANT = 1;" +
"var x = goog.CONSTANT;",
"(function() {})('goog.CONSTANT', 1);" +
"var x = 1;");
}
public void testBug2410122() {
CompilerOptions options = createCompilerOptions();
options.generateExports = true;
options.closurePass = true;
test(options,
"var goog = {};" +
"function F() {}" +
"/** @export */ function G() { goog.base(this); } " +
"goog.inherits(G, F);",
"var goog = {};" +
"function F() {}" +
"function G() { F.call(this); } " +
"goog.inherits(G, F); goog.exportSymbol('G', G);");
}
public void testIssue90() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
options.inlineVariables = true;
options.removeDeadCode = true;
test(options,
"var x; x && alert(1);",
"");
}
public void testClosurePassOff() {
CompilerOptions options = createCompilerOptions();
options.closurePass = false;
testSame(
options,
"var goog = {}; goog.require = function(x) {}; goog.require('foo');");
testSame(
options,
"var goog = {}; goog.getCssName = function(x) {};" +
"goog.getCssName('foo');");
}
public void testClosurePassOn() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
test(
options,
"var goog = {}; goog.require = function(x) {}; goog.require('foo');",
ProcessClosurePrimitives.MISSING_PROVIDE_ERROR);
test(
options,
"/** @define {boolean} */ var COMPILED = false;" +
"var goog = {}; goog.getCssName = function(x) {};" +
"goog.getCssName('foo');",
"var COMPILED = true;" +
"var goog = {}; goog.getCssName = function(x) {};" +
"'foo';");
}
public void testCssNameCheck() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.checkMissingGetCssNameLevel = CheckLevel.ERROR;
options.checkMissingGetCssNameBlacklist = "foo";
test(options, "var x = 'foo';",
CheckMissingGetCssName.MISSING_GETCSSNAME);
}
public void testBug2592659() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.checkTypes = true;
options.checkMissingGetCssNameLevel = CheckLevel.WARNING;
options.checkMissingGetCssNameBlacklist = "foo";
test(options,
"var goog = {};\n" +
"/**\n" +
" * @param {string} className\n" +
" * @param {string=} opt_modifier\n" +
" * @return {string}\n" +
"*/\n" +
"goog.getCssName = function(className, opt_modifier) {}\n" +
"var x = goog.getCssName(123, 'a');",
TypeValidator.TYPE_MISMATCH_WARNING);
}
public void testTypedefBeforeOwner1() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
test(options,
"goog.provide('foo.Bar.Type');\n" +
"goog.provide('foo.Bar');\n" +
"/** @typedef {number} */ foo.Bar.Type;\n" +
"foo.Bar = function() {};",
"var foo = {}; foo.Bar.Type; foo.Bar = function() {};");
}
public void testTypedefBeforeOwner2() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.collapseProperties = true;
test(options,
"goog.provide('foo.Bar.Type');\n" +
"goog.provide('foo.Bar');\n" +
"/** @typedef {number} */ foo.Bar.Type;\n" +
"foo.Bar = function() {};",
"var foo$Bar$Type; var foo$Bar = function() {};");
}
public void testExportedNames() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.variableRenaming = VariableRenamingPolicy.ALL;
test(options,
"/** @define {boolean} */ var COMPILED = false;" +
"var goog = {}; goog.exportSymbol('b', goog);",
"var a = true; var c = {}; c.exportSymbol('b', c);");
test(options,
"/** @define {boolean} */ var COMPILED = false;" +
"var goog = {}; goog.exportSymbol('a', goog);",
"var b = true; var c = {}; c.exportSymbol('a', c);");
}
public void testCheckGlobalThisOn() {
CompilerOptions options = createCompilerOptions();
options.checkSuspiciousCode = true;
options.checkGlobalThisLevel = CheckLevel.ERROR;
test(options, "function f() { this.y = 3; }", CheckGlobalThis.GLOBAL_THIS);
}
public void testSusiciousCodeOff() {
CompilerOptions options = createCompilerOptions();
options.checkSuspiciousCode = false;
options.checkGlobalThisLevel = CheckLevel.ERROR;
test(options, "function f() { this.y = 3; }", CheckGlobalThis.GLOBAL_THIS);
}
public void testCheckGlobalThisOff() {
CompilerOptions options = createCompilerOptions();
options.checkSuspiciousCode = true;
options.checkGlobalThisLevel = CheckLevel.OFF;
testSame(options, "function f() { this.y = 3; }");
}
public void testCheckRequiresAndCheckProvidesOff() {
testSame(createCompilerOptions(), new String[] {
"/** @constructor */ function Foo() {}",
"new Foo();"
});
}
public void testCheckRequiresOn() {
CompilerOptions options = createCompilerOptions();
options.checkRequires = CheckLevel.ERROR;
test(options, new String[] {
"/** @constructor */ function Foo() {}",
"new Foo();"
}, CheckRequiresForConstructors.MISSING_REQUIRE_WARNING);
}
public void testCheckProvidesOn() {
CompilerOptions options = createCompilerOptions();
options.checkProvides = CheckLevel.ERROR;
test(options, new String[] {
"/** @constructor */ function Foo() {}",
"new Foo();"
}, CheckProvides.MISSING_PROVIDE_WARNING);
}
public void testGenerateExportsOff() {
testSame(createCompilerOptions(), "/** @export */ function f() {}");
}
public void testGenerateExportsOn() {
CompilerOptions options = createCompilerOptions();
options.generateExports = true;
test(options, "/** @export */ function f() {}",
"/** @export */ function f() {} goog.exportSymbol('f', f);");
}
public void testExportTestFunctionsOff() {
testSame(createCompilerOptions(), "function testFoo() {}");
}
public void testExportTestFunctionsOn() {
CompilerOptions options = createCompilerOptions();
options.exportTestFunctions = true;
test(options, "function testFoo() {}",
"/** @export */ function testFoo() {}" +
"goog.exportSymbol('testFoo', testFoo);");
}
public void testExpose() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.ADVANCED_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
test(options,
"var x = {eeny: 1, /** @expose */ meeny: 2};" +
"/** @constructor */ var Foo = function() {};" +
"/** @expose */ Foo.prototype.miny = 3;" +
"Foo.prototype.moe = 4;" +
"function moe(a, b) { return a.meeny + b.miny; }" +
"window['x'] = x;" +
"window['Foo'] = Foo;" +
"window['moe'] = moe;",
"function a(){}" +
"a.prototype.miny=3;" +
"window.x={a:1,meeny:2};" +
"window.Foo=a;" +
"window.moe=function(b,c){" +
" return b.meeny+c.miny" +
"}");
}
public void testCheckSymbolsOff() {
CompilerOptions options = createCompilerOptions();
testSame(options, "x = 3;");
}
public void testCheckSymbolsOn() {
CompilerOptions options = createCompilerOptions();
options.checkSymbols = true;
test(options, "x = 3;", VarCheck.UNDEFINED_VAR_ERROR);
}
public void testCheckReferencesOff() {
CompilerOptions options = createCompilerOptions();
testSame(options, "x = 3; var x = 5;");
}
public void testCheckReferencesOn() {
CompilerOptions options = createCompilerOptions();
options.aggressiveVarCheck = CheckLevel.ERROR;
test(options, "x = 3; var x = 5;",
VariableReferenceCheck.UNDECLARED_REFERENCE);
}
public void testInferTypes() {
CompilerOptions options = createCompilerOptions();
options.inferTypes = true;
options.checkTypes = false;
options.closurePass = true;
test(options,
CLOSURE_BOILERPLATE +
"goog.provide('Foo'); /** @enum */ Foo = {a: 3};",
TypeCheck.ENUM_NOT_CONSTANT);
assertTrue(lastCompiler.getErrorManager().getTypedPercent() == 0);
// This does not generate a warning.
test(options, "/** @type {number} */ var n = window.name;",
"var n = window.name;");
assertTrue(lastCompiler.getErrorManager().getTypedPercent() == 0);
}
public void testTypeCheckAndInference() {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
test(options, "/** @type {number} */ var n = window.name;",
TypeValidator.TYPE_MISMATCH_WARNING);
assertTrue(lastCompiler.getErrorManager().getTypedPercent() > 0);
}
public void testTypeNameParser() {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
test(options, "/** @type {n} */ var n = window.name;",
RhinoErrorReporter.TYPE_PARSE_ERROR);
}
// This tests that the TypedScopeCreator is memoized so that it only creates a
// Scope object once for each scope. If, when type inference requests a scope,
// it creates a new one, then multiple JSType objects end up getting created
// for the same local type, and ambiguate will rename the last statement to
// o.a(o.a, o.a), which is bad.
public void testMemoizedTypedScopeCreator() {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
options.ambiguateProperties = true;
options.propertyRenaming = PropertyRenamingPolicy.ALL_UNQUOTED;
test(options, "function someTest() {\n"
+ " /** @constructor */\n"
+ " function Foo() { this.instProp = 3; }\n"
+ " Foo.prototype.protoProp = function(a, b) {};\n"
+ " /** @constructor\n @extends Foo */\n"
+ " function Bar() {}\n"
+ " goog.inherits(Bar, Foo);\n"
+ " var o = new Bar();\n"
+ " o.protoProp(o.protoProp, o.instProp);\n"
+ "}",
"function someTest() {\n"
+ " function Foo() { this.b = 3; }\n"
+ " Foo.prototype.a = function(a, b) {};\n"
+ " function Bar() {}\n"
+ " goog.c(Bar, Foo);\n"
+ " var o = new Bar();\n"
+ " o.a(o.a, o.b);\n"
+ "}");
}
public void testCheckTypes() {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
test(options, "var x = x || {}; x.f = function() {}; x.f(3);",
TypeCheck.WRONG_ARGUMENT_COUNT);
}
public void testReplaceCssNames() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.gatherCssNames = true;
test(options, "/** @define {boolean} */\n"
+ "var COMPILED = false;\n"
+ "goog.setCssNameMapping({'foo':'bar'});\n"
+ "function getCss() {\n"
+ " return goog.getCssName('foo');\n"
+ "}",
"var COMPILED = true;\n"
+ "function getCss() {\n"
+ " return \"bar\";"
+ "}");
assertEquals(
ImmutableMap.of("foo", new Integer(1)),
lastCompiler.getPassConfig().getIntermediateState().cssNames);
}
public void testRemoveClosureAsserts() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
testSame(options,
"var goog = {};"
+ "goog.asserts.assert(goog);");
options.removeClosureAsserts = true;
test(options,
"var goog = {};"
+ "goog.asserts.assert(goog);",
"var goog = {};");
}
public void testDeprecation() {
String code = "/** @deprecated */ function f() { } function g() { f(); }";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.setWarningLevel(DiagnosticGroups.DEPRECATED, CheckLevel.ERROR);
testSame(options, code);
options.checkTypes = true;
test(options, code, CheckAccessControls.DEPRECATED_NAME);
}
public void testVisibility() {
String[] code = {
"/** @private */ function f() { }",
"function g() { f(); }"
};
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.setWarningLevel(DiagnosticGroups.VISIBILITY, CheckLevel.ERROR);
testSame(options, code);
options.checkTypes = true;
test(options, code, CheckAccessControls.BAD_PRIVATE_GLOBAL_ACCESS);
}
public void testUnreachableCode() {
String code = "function f() { return \n 3; }";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.checkUnreachableCode = CheckLevel.ERROR;
test(options, code, CheckUnreachableCode.UNREACHABLE_CODE);
}
public void testMissingReturn() {
String code =
"/** @return {number} */ function f() { if (f) { return 3; } }";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.checkMissingReturn = CheckLevel.ERROR;
testSame(options, code);
options.checkTypes = true;
test(options, code, CheckMissingReturn.MISSING_RETURN_STATEMENT);
}
public void testIdGenerators() {
String code = "function f() {} f('id');";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.idGenerators = Sets.newHashSet("f");
test(options, code, "function f() {} 'a';");
}
public void testOptimizeArgumentsArray() {
String code = "function f() { return arguments[0]; }";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.optimizeArgumentsArray = true;
String argName = "JSCompiler_OptimizeArgumentsArray_p0";
test(options, code,
"function f(" + argName + ") { return " + argName + "; }");
}
public void testOptimizeParameters() {
String code = "function f(a) { return a; } f(true);";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.optimizeParameters = true;
test(options, code, "function f() { var a = true; return a;} f();");
}
public void testOptimizeReturns() {
String code = "function f(a) { return a; } f(true);";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.optimizeReturns = true;
test(options, code, "function f(a) {return;} f(true);");
}
public void testRemoveAbstractMethods() {
String code = CLOSURE_BOILERPLATE +
"var x = {}; x.foo = goog.abstractMethod; x.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.closurePass = true;
options.collapseProperties = true;
test(options, code, CLOSURE_COMPILED + " var x$bar = 3;");
}
public void testCollapseProperties1() {
String code =
"var x = {}; x.FOO = 5; x.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.collapseProperties = true;
test(options, code, "var x$FOO = 5; var x$bar = 3;");
}
public void testCollapseProperties2() {
String code =
"var x = {}; x.FOO = 5; x.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.collapseProperties = true;
options.collapseObjectLiterals = true;
test(options, code, "var x$FOO = 5; var x$bar = 3;");
}
public void testCollapseObjectLiteral1() {
// Verify collapseObjectLiterals does nothing in global scope
String code = "var x = {}; x.FOO = 5; x.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.collapseObjectLiterals = true;
testSame(options, code);
}
public void testCollapseObjectLiteral2() {
String code =
"function f() {var x = {}; x.FOO = 5; x.bar = 3;}";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.collapseObjectLiterals = true;
test(options, code,
"function f(){" +
"var JSCompiler_object_inline_FOO_0;" +
"var JSCompiler_object_inline_bar_1;" +
"JSCompiler_object_inline_FOO_0=5;" +
"JSCompiler_object_inline_bar_1=3}");
}
public void testTightenTypesWithoutTypeCheck() {
CompilerOptions options = createCompilerOptions();
options.tightenTypes = true;
test(options, "", DefaultPassConfig.TIGHTEN_TYPES_WITHOUT_TYPE_CHECK);
}
public void testDisambiguateProperties() {
String code =
"/** @constructor */ function Foo(){} Foo.prototype.bar = 3;" +
"/** @constructor */ function Baz(){} Baz.prototype.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.disambiguateProperties = true;
options.checkTypes = true;
test(options, code,
"function Foo(){} Foo.prototype.Foo_prototype$bar = 3;" +
"function Baz(){} Baz.prototype.Baz_prototype$bar = 3;");
}
public void testMarkPureCalls() {
String testCode = "function foo() {} foo();";
CompilerOptions options = createCompilerOptions();
options.removeDeadCode = true;
testSame(options, testCode);
options.computeFunctionSideEffects = true;
test(options, testCode, "function foo() {}");
}
public void testMarkNoSideEffects() {
String testCode = "noSideEffects();";
CompilerOptions options = createCompilerOptions();
options.removeDeadCode = true;
testSame(options, testCode);
options.markNoSideEffectCalls = true;
test(options, testCode, "");
}
public void testChainedCalls() {
CompilerOptions options = createCompilerOptions();
options.chainCalls = true;
test(
options,
"/** @constructor */ function Foo() {} " +
"Foo.prototype.bar = function() { return this; }; " +
"var f = new Foo();" +
"f.bar(); " +
"f.bar(); ",
"function Foo() {} " +
"Foo.prototype.bar = function() { return this; }; " +
"var f = new Foo();" +
"f.bar().bar();");
}
public void testExtraAnnotationNames() {
CompilerOptions options = createCompilerOptions();
options.setExtraAnnotationNames(Sets.newHashSet("TagA", "TagB"));
test(
options,
"/** @TagA */ var f = new Foo(); /** @TagB */ f.bar();",
"var f = new Foo(); f.bar();");
}
public void testDevirtualizePrototypeMethods() {
CompilerOptions options = createCompilerOptions();
options.devirtualizePrototypeMethods = true;
test(
options,
"/** @constructor */ var Foo = function() {}; " +
"Foo.prototype.bar = function() {};" +
"(new Foo()).bar();",
"var Foo = function() {};" +
"var JSCompiler_StaticMethods_bar = " +
" function(JSCompiler_StaticMethods_bar$self) {};" +
"JSCompiler_StaticMethods_bar(new Foo());");
}
public void testCheckConsts() {
CompilerOptions options = createCompilerOptions();
options.inlineConstantVars = true;
test(options, "var FOO = true; FOO = false",
ConstCheck.CONST_REASSIGNED_VALUE_ERROR);
}
public void testAllChecksOn() {
CompilerOptions options = createCompilerOptions();
options.checkSuspiciousCode = true;
options.checkControlStructures = true;
options.checkRequires = CheckLevel.ERROR;
options.checkProvides = CheckLevel.ERROR;
options.generateExports = true;
options.exportTestFunctions = true;
options.closurePass = true;
options.checkMissingGetCssNameLevel = CheckLevel.ERROR;
options.checkMissingGetCssNameBlacklist = "goog";
options.syntheticBlockStartMarker = "synStart";
options.syntheticBlockEndMarker = "synEnd";
options.checkSymbols = true;
options.aggressiveVarCheck = CheckLevel.ERROR;
options.processObjectPropertyString = true;
options.collapseProperties = true;
test(options, CLOSURE_BOILERPLATE, CLOSURE_COMPILED);
}
public void testTypeCheckingWithSyntheticBlocks() {
CompilerOptions options = createCompilerOptions();
options.syntheticBlockStartMarker = "synStart";
options.syntheticBlockEndMarker = "synEnd";
options.checkTypes = true;
// We used to have a bug where the CFG drew an
// edge straight from synStart to f(progress).
// If that happens, then progress will get type {number|undefined}.
testSame(
options,
"/** @param {number} x */ function f(x) {}" +
"function g() {" +
" synStart('foo');" +
" var progress = 1;" +
" f(progress);" +
" synEnd('foo');" +
"}");
}
public void testCompilerDoesNotBlowUpIfUndefinedSymbols() {
CompilerOptions options = createCompilerOptions();
options.checkSymbols = true;
// Disable the undefined variable check.
options.setWarningLevel(
DiagnosticGroup.forType(VarCheck.UNDEFINED_VAR_ERROR),
CheckLevel.OFF);
// The compiler used to throw an IllegalStateException on this.
testSame(options, "var x = {foo: y};");
}
// Make sure that if we change variables which are constant to have
// $$constant appended to their names, we remove that tag before
// we finish.
public void testConstantTagsMustAlwaysBeRemoved() {
CompilerOptions options = createCompilerOptions();
options.variableRenaming = VariableRenamingPolicy.LOCAL;
String originalText = "var G_GEO_UNKNOWN_ADDRESS=1;\n" +
"function foo() {" +
" var localVar = 2;\n" +
" if (G_GEO_UNKNOWN_ADDRESS == localVar) {\n" +
" alert(\"A\"); }}";
String expectedText = "var G_GEO_UNKNOWN_ADDRESS=1;" +
"function foo(){var a=2;if(G_GEO_UNKNOWN_ADDRESS==a){alert(\"A\")}}";
test(options, originalText, expectedText);
}
public void testClosurePassPreservesJsDoc() {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
options.closurePass = true;
test(options,
CLOSURE_BOILERPLATE +
"goog.provide('Foo'); /** @constructor */ Foo = function() {};" +
"var x = new Foo();",
"var COMPILED=true;var goog={};goog.exportSymbol=function(){};" +
"var Foo=function(){};var x=new Foo");
test(options,
CLOSURE_BOILERPLATE +
"goog.provide('Foo'); /** @enum */ Foo = {a: 3};",
TypeCheck.ENUM_NOT_CONSTANT);
}
public void testProvidedNamespaceIsConst() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.inlineConstantVars = true;
options.collapseProperties = true;
test(options,
"var goog = {}; goog.provide('foo'); " +
"function f() { foo = {};}",
"var foo = {}; function f() { foo = {}; }",
ConstCheck.CONST_REASSIGNED_VALUE_ERROR);
}
public void testProvidedNamespaceIsConst2() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.inlineConstantVars = true;
options.collapseProperties = true;
test(options,
"var goog = {}; goog.provide('foo.bar'); " +
"function f() { foo.bar = {};}",
"var foo$bar = {};" +
"function f() { foo$bar = {}; }",
ConstCheck.CONST_REASSIGNED_VALUE_ERROR);
}
public void testProvidedNamespaceIsConst3() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.inlineConstantVars = true;
options.collapseProperties = true;
test(options,
"var goog = {}; " +
"goog.provide('foo.bar'); goog.provide('foo.bar.baz'); " +
"/** @constructor */ foo.bar = function() {};" +
"/** @constructor */ foo.bar.baz = function() {};",
"var foo$bar = function(){};" +
"var foo$bar$baz = function(){};");
}
public void testProvidedNamespaceIsConst4() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.inlineConstantVars = true;
options.collapseProperties = true;
test(options,
"var goog = {}; goog.provide('foo.Bar'); " +
"var foo = {}; foo.Bar = {};",
"var foo = {}; var foo = {}; foo.Bar = {};");
}
public void testProvidedNamespaceIsConst5() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.inlineConstantVars = true;
options.collapseProperties = true;
test(options,
"var goog = {}; goog.provide('foo.Bar'); " +
"foo = {}; foo.Bar = {};",
"var foo = {}; foo = {}; foo.Bar = {};");
}
public void testProcessDefinesAlwaysOn() {
test(createCompilerOptions(),
"/** @define {boolean} */ var HI = true; HI = false;",
"var HI = false;false;");
}
public void testProcessDefinesAdditionalReplacements() {
CompilerOptions options = createCompilerOptions();
options.setDefineToBooleanLiteral("HI", false);
test(options,
"/** @define {boolean} */ var HI = true;",
"var HI = false;");
}
public void testReplaceMessages() {
CompilerOptions options = createCompilerOptions();
String prefix = "var goog = {}; goog.getMsg = function() {};";
testSame(options, prefix + "var MSG_HI = goog.getMsg('hi');");
options.messageBundle = new EmptyMessageBundle();
test(options,
prefix + "/** @desc xyz */ var MSG_HI = goog.getMsg('hi');",
prefix + "var MSG_HI = 'hi';");
}
public void testCheckGlobalNames() {
CompilerOptions options = createCompilerOptions();
options.checkGlobalNamesLevel = CheckLevel.ERROR;
test(options, "var x = {}; var y = x.z;",
CheckGlobalNames.UNDEFINED_NAME_WARNING);
}
public void testInlineGetters() {
CompilerOptions options = createCompilerOptions();
String code =
"function Foo() {} Foo.prototype.bar = function() { return 3; };" +
"var x = new Foo(); x.bar();";
testSame(options, code);
options.inlineGetters = true;
test(options, code,
"function Foo() {} Foo.prototype.bar = function() { return 3 };" +
"var x = new Foo(); 3;");
}
public void testInlineGettersWithAmbiguate() {
CompilerOptions options = createCompilerOptions();
String code =
"/** @constructor */" +
"function Foo() {}" +
"/** @type {number} */ Foo.prototype.field;" +
"Foo.prototype.getField = function() { return this.field; };" +
"/** @constructor */" +
"function Bar() {}" +
"/** @type {string} */ Bar.prototype.field;" +
"Bar.prototype.getField = function() { return this.field; };" +
"new Foo().getField();" +
"new Bar().getField();";
testSame(options, code);
options.inlineGetters = true;
test(options, code,
"function Foo() {}" +
"Foo.prototype.field;" +
"Foo.prototype.getField = function() { return this.field; };" +
"function Bar() {}" +
"Bar.prototype.field;" +
"Bar.prototype.getField = function() { return this.field; };" +
"new Foo().field;" +
"new Bar().field;");
options.checkTypes = true;
options.ambiguateProperties = true;
// Propagating the wrong type information may cause ambiguate properties
// to generate bad code.
testSame(options, code);
}
public void testInlineVariables() {
CompilerOptions options = createCompilerOptions();
String code = "function foo() {} var x = 3; foo(x);";
testSame(options, code);
options.inlineVariables = true;
test(options, code, "(function foo() {})(3);");
options.propertyRenaming = PropertyRenamingPolicy.HEURISTIC;
test(options, code, DefaultPassConfig.CANNOT_USE_PROTOTYPE_AND_VAR);
}
public void testInlineConstants() {
CompilerOptions options = createCompilerOptions();
String code = "function foo() {} var x = 3; foo(x); var YYY = 4; foo(YYY);";
testSame(options, code);
options.inlineConstantVars = true;
test(options, code, "function foo() {} var x = 3; foo(x); foo(4);");
}
public void testMinimizeExits() {
CompilerOptions options = createCompilerOptions();
String code =
"function f() {" +
" if (window.foo) return; window.h(); " +
"}";
testSame(options, code);
options.foldConstants = true;
test(
options, code,
"function f() {" +
" window.foo || window.h(); " +
"}");
}
public void testFoldConstants() {
CompilerOptions options = createCompilerOptions();
String code = "if (true) { window.foo(); }";
testSame(options, code);
options.foldConstants = true;
test(options, code, "window.foo();");
}
public void testRemoveUnreachableCode() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { return; f(); }";
testSame(options, code);
options.removeDeadCode = true;
test(options, code, "function f() {}");
}
public void testRemoveUnusedPrototypeProperties1() {
CompilerOptions options = createCompilerOptions();
String code = "function Foo() {} " +
"Foo.prototype.bar = function() { return new Foo(); };";
testSame(options, code);
options.removeUnusedPrototypeProperties = true;
test(options, code, "function Foo() {}");
}
public void testRemoveUnusedPrototypeProperties2() {
CompilerOptions options = createCompilerOptions();
String code = "function Foo() {} " +
"Foo.prototype.bar = function() { return new Foo(); };" +
"function f(x) { x.bar(); }";
testSame(options, code);
options.removeUnusedPrototypeProperties = true;
testSame(options, code);
options.removeUnusedVars = true;
test(options, code, "");
}
public void testSmartNamePass() {
CompilerOptions options = createCompilerOptions();
String code = "function Foo() { this.bar(); } " +
"Foo.prototype.bar = function() { return Foo(); };";
testSame(options, code);
options.smartNameRemoval = true;
test(options, code, "");
}
public void testDeadAssignmentsElimination() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { var x = 3; 4; x = 5; return x; } f(); ";
testSame(options, code);
options.deadAssignmentElimination = true;
testSame(options, code);
options.removeUnusedVars = true;
test(options, code, "function f() { var x = 3; 4; x = 5; return x; } f();");
}
public void testInlineFunctions() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { return 3; } f(); ";
testSame(options, code);
options.inlineFunctions = true;
test(options, code, "3;");
}
public void testRemoveUnusedVars1() {
CompilerOptions options = createCompilerOptions();
String code = "function f(x) {} f();";
testSame(options, code);
options.removeUnusedVars = true;
test(options, code, "function f() {} f();");
}
public void testRemoveUnusedVars2() {
CompilerOptions options = createCompilerOptions();
String code = "(function f(x) {})();var g = function() {}; g();";
testSame(options, code);
options.removeUnusedVars = true;
test(options, code, "(function() {})();var g = function() {}; g();");
options.anonymousFunctionNaming = AnonymousFunctionNamingPolicy.UNMAPPED;
test(options, code, "(function f() {})();var g = function $g$() {}; g();");
}
public void testCrossModuleCodeMotion() {
CompilerOptions options = createCompilerOptions();
String[] code = new String[] {
"var x = 1;",
"x;",
};
testSame(options, code);
options.crossModuleCodeMotion = true;
test(options, code, new String[] {
"",
"var x = 1; x;",
});
}
public void testCrossModuleMethodMotion() {
CompilerOptions options = createCompilerOptions();
String[] code = new String[] {
"var Foo = function() {}; Foo.prototype.bar = function() {};" +
"var x = new Foo();",
"x.bar();",
};
testSame(options, code);
options.crossModuleMethodMotion = true;
test(options, code, new String[] {
CrossModuleMethodMotion.STUB_DECLARATIONS +
"var Foo = function() {};" +
"Foo.prototype.bar=JSCompiler_stubMethod(0); var x=new Foo;",
"Foo.prototype.bar=JSCompiler_unstubMethod(0,function(){}); x.bar()",
});
}
public void testFlowSensitiveInlineVariables1() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { var x = 3; x = 5; return x; }";
testSame(options, code);
options.flowSensitiveInlineVariables = true;
test(options, code, "function f() { var x = 3; return 5; }");
String unusedVar = "function f() { var x; x = 5; return x; } f()";
test(options, unusedVar, "function f() { var x; return 5; } f()");
options.removeUnusedVars = true;
test(options, unusedVar, "function f() { return 5; } f()");
}
public void testFlowSensitiveInlineVariables2() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.SIMPLE_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
test(options,
"function f () {\n" +
" var ab = 0;\n" +
" ab += '-';\n" +
" alert(ab);\n" +
"}",
"function f () {\n" +
" alert('0-');\n" +
"}");
}
public void testCollapseAnonymousFunctions() {
CompilerOptions options = createCompilerOptions();
String code = "var f = function() {};";
testSame(options, code);
options.collapseAnonymousFunctions = true;
test(options, code, "function f() {}");
}
public void testMoveFunctionDeclarations() {
CompilerOptions options = createCompilerOptions();
String code = "var x = f(); function f() { return 3; }";
testSame(options, code);
options.moveFunctionDeclarations = true;
test(options, code, "function f() { return 3; } var x = f();");
}
public void testNameAnonymousFunctions() {
CompilerOptions options = createCompilerOptions();
String code = "var f = function() {};";
testSame(options, code);
options.anonymousFunctionNaming = AnonymousFunctionNamingPolicy.MAPPED;
test(options, code, "var f = function $() {}");
assertNotNull(lastCompiler.getResult().namedAnonFunctionMap);
options.anonymousFunctionNaming = AnonymousFunctionNamingPolicy.UNMAPPED;
test(options, code, "var f = function $f$() {}");
assertNull(lastCompiler.getResult().namedAnonFunctionMap);
}
public void testNameAnonymousFunctionsWithVarRemoval() {
CompilerOptions options = createCompilerOptions();
options.setRemoveUnusedVariables(CompilerOptions.Reach.LOCAL_ONLY);
options.setInlineVariables(true);
String code = "var f = function longName() {}; var g = function() {};" +
"function longerName() {} var i = longerName;";
test(options, code,
"var f = function() {}; var g = function() {}; " +
"var i = function() {};");
options.anonymousFunctionNaming = AnonymousFunctionNamingPolicy.MAPPED;
test(options, code,
"var f = function longName() {}; var g = function $() {};" +
"var i = function longerName(){};");
assertNotNull(lastCompiler.getResult().namedAnonFunctionMap);
options.anonymousFunctionNaming = AnonymousFunctionNamingPolicy.UNMAPPED;
test(options, code,
"var f = function longName() {}; var g = function $g$() {};" +
"var i = function longerName(){};");
assertNull(lastCompiler.getResult().namedAnonFunctionMap);
}
public void testExtractPrototypeMemberDeclarations() {
CompilerOptions options = createCompilerOptions();
String code = "var f = function() {};";
String expected = "var a; var b = function() {}; a = b.prototype;";
for (int i = 0; i < 10; i++) {
code += "f.prototype.a = " + i + ";";
expected += "a.a = " + i + ";";
}
testSame(options, code);
options.extractPrototypeMemberDeclarations = true;
options.variableRenaming = VariableRenamingPolicy.ALL;
test(options, code, expected);
options.propertyRenaming = PropertyRenamingPolicy.HEURISTIC;
options.variableRenaming = VariableRenamingPolicy.OFF;
testSame(options, code);
}
public void testDevirtualizationAndExtractPrototypeMemberDeclarations() {
CompilerOptions options = createCompilerOptions();
options.devirtualizePrototypeMethods = true;
options.collapseAnonymousFunctions = true;
options.extractPrototypeMemberDeclarations = true;
options.variableRenaming = VariableRenamingPolicy.ALL;
String code = "var f = function() {};";
String expected = "var a; function b() {} a = b.prototype;";
for (int i = 0; i < 10; i++) {
code += "f.prototype.argz = function() {arguments};";
code += "f.prototype.devir" + i + " = function() {};";
char letter = (char) ('d' + i);
expected += "a.argz = function() {arguments};";
expected += "function " + letter + "(c){}";
}
code += "var F = new f(); F.argz();";
expected += "var n = new b(); n.argz();";
for (int i = 0; i < 10; i++) {
code += "F.devir" + i + "();";
char letter = (char) ('d' + i);
expected += letter + "(n);";
}
test(options, code, expected);
}
public void testCoalesceVariableNames() {
CompilerOptions options = createCompilerOptions();
String code = "function f() {var x = 3; var y = x; var z = y; return z;}";
testSame(options, code);
options.coalesceVariableNames = true;
test(options, code,
"function f() {var x = 3; x = x; x = x; return x;}");
}
public void testPropertyRenaming() {
CompilerOptions options = createCompilerOptions();
options.propertyAffinity = true;
String code =
"function f() { return this.foo + this['bar'] + this.Baz; }" +
"f.prototype.bar = 3; f.prototype.Baz = 3;";
String heuristic =
"function f() { return this.foo + this['bar'] + this.a; }" +
"f.prototype.bar = 3; f.prototype.a = 3;";
String aggHeuristic =
"function f() { return this.foo + this['b'] + this.a; } " +
"f.prototype.b = 3; f.prototype.a = 3;";
String all =
"function f() { return this.b + this['bar'] + this.a; }" +
"f.prototype.c = 3; f.prototype.a = 3;";
testSame(options, code);
options.propertyRenaming = PropertyRenamingPolicy.HEURISTIC;
test(options, code, heuristic);
options.propertyRenaming = PropertyRenamingPolicy.AGGRESSIVE_HEURISTIC;
test(options, code, aggHeuristic);
options.propertyRenaming = PropertyRenamingPolicy.ALL_UNQUOTED;
test(options, code, all);
}
public void testConvertToDottedProperties() {
CompilerOptions options = createCompilerOptions();
String code =
"function f() { return this['bar']; } f.prototype.bar = 3;";
String expected =
"function f() { return this.bar; } f.prototype.a = 3;";
testSame(options, code);
options.convertToDottedProperties = true;
options.propertyRenaming = PropertyRenamingPolicy.ALL_UNQUOTED;
test(options, code, expected);
}
public void testRewriteFunctionExpressions() {
CompilerOptions options = createCompilerOptions();
String code = "var a = function() {};";
String expected = "function JSCompiler_emptyFn(){return function(){}} " +
"var a = JSCompiler_emptyFn();";
for (int i = 0; i < 10; i++) {
code += "a = function() {};";
expected += "a = JSCompiler_emptyFn();";
}
testSame(options, code);
options.rewriteFunctionExpressions = true;
test(options, code, expected);
}
public void testAliasAllStrings() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { return 'a'; }";
String expected = "var $$S_a = 'a'; function f() { return $$S_a; }";
testSame(options, code);
options.aliasAllStrings = true;
test(options, code, expected);
}
public void testAliasExterns() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { return window + window + window + window; }";
String expected = "var GLOBAL_window = window;" +
"function f() { return GLOBAL_window + GLOBAL_window + " +
" GLOBAL_window + GLOBAL_window; }";
testSame(options, code);
options.aliasExternals = true;
test(options, code, expected);
}
public void testAliasKeywords() {
CompilerOptions options = createCompilerOptions();
String code =
"function f() { return true + true + true + true + true + true; }";
String expected = "var JSCompiler_alias_TRUE = true;" +
"function f() { return JSCompiler_alias_TRUE + " +
" JSCompiler_alias_TRUE + JSCompiler_alias_TRUE + " +
" JSCompiler_alias_TRUE + JSCompiler_alias_TRUE + " +
" JSCompiler_alias_TRUE; }";
testSame(options, code);
options.aliasKeywords = true;
test(options, code, expected);
}
public void testRenameVars1() {
CompilerOptions options = createCompilerOptions();
String code =
"var abc = 3; function f() { var xyz = 5; return abc + xyz; }";
String local = "var abc = 3; function f() { var a = 5; return abc + a; }";
String all = "var a = 3; function c() { var b = 5; return a + b; }";
testSame(options, code);
options.variableRenaming = VariableRenamingPolicy.LOCAL;
test(options, code, local);
options.variableRenaming = VariableRenamingPolicy.ALL;
test(options, code, all);
options.reserveRawExports = true;
}
public void testRenameVars2() {
CompilerOptions options = createCompilerOptions();
options.variableRenaming = VariableRenamingPolicy.ALL;
String code = "var abc = 3; function f() { window['a'] = 5; }";
String noexport = "var a = 3; function b() { window['a'] = 5; }";
String export = "var b = 3; function c() { window['a'] = 5; }";
options.reserveRawExports = false;
test(options, code, noexport);
options.reserveRawExports = true;
test(options, code, export);
}
public void testShadowVaribles() {
CompilerOptions options = createCompilerOptions();
options.variableRenaming = VariableRenamingPolicy.LOCAL;
options.shadowVariables = true;
String code = "var f = function(x) { return function(y) {}}";
String expected = "var f = function(a) { return function(a) {}}";
test(options, code, expected);
}
public void testRenameLabels() {
CompilerOptions options = createCompilerOptions();
String code = "longLabel: while (true) { break longLabel; }";
String expected = "a: while (true) { break a; }";
testSame(options, code);
options.labelRenaming = true;
test(options, code, expected);
}
public void testBadBreakStatementInIdeMode() {
// Ensure that type-checking doesn't crash, even if the CFG is malformed.
// This can happen in IDE mode.
CompilerOptions options = createCompilerOptions();
options.ideMode = true;
options.checkTypes = true;
test(options,
"function f() { try { } catch(e) { break; } }",
RhinoErrorReporter.PARSE_ERROR);
}
public void testIssue63SourceMap() {
CompilerOptions options = createCompilerOptions();
String code = "var a;";
options.skipAllPasses = true;
options.sourceMapOutputPath = "./src.map";
Compiler compiler = compile(options, code);
compiler.toSource();
}
public void testRegExp1() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = "/(a)/.test(\"a\");";
testSame(options, code);
options.computeFunctionSideEffects = true;
String expected = "";
test(options, code, expected);
}
public void testRegExp2() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = "/(a)/.test(\"a\");var a = RegExp.$1";
testSame(options, code);
options.computeFunctionSideEffects = true;
test(options, code, CheckRegExp.REGEXP_REFERENCE);
options.setWarningLevel(DiagnosticGroups.CHECK_REGEXP, CheckLevel.OFF);
testSame(options, code);
}
public void testFoldLocals1() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
// An external object, whose constructor has no side-effects,
// and whose method "go" only modifies the object.
String code = "new Widget().go();";
testSame(options, code);
options.computeFunctionSideEffects = true;
test(options, code, "");
}
public void testFoldLocals2() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
options.checkTypes = true;
// An external function that returns a local object that the
// method "go" that only modifies the object.
String code = "widgetToken().go();";
testSame(options, code);
options.computeFunctionSideEffects = true;
test(options, code, "widgetToken()");
}
public void testFoldLocals3() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
// A function "f" who returns a known local object, and a method that
// modifies only modifies that.
String definition = "function f(){return new Widget()}";
String call = "f().go();";
String code = definition + call;
testSame(options, code);
options.computeFunctionSideEffects = true;
// BROKEN
//test(options, code, definition);
testSame(options, code);
}
public void testFoldLocals4() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = "/** @constructor */\n"
+ "function InternalWidget(){this.x = 1;}"
+ "InternalWidget.prototype.internalGo = function (){this.x = 2};"
+ "new InternalWidget().internalGo();";
testSame(options, code);
options.computeFunctionSideEffects = true;
String optimized = ""
+ "function InternalWidget(){this.x = 1;}"
+ "InternalWidget.prototype.internalGo = function (){this.x = 2};";
test(options, code, optimized);
}
public void testFoldLocals5() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = ""
+ "function fn(){var a={};a.x={};return a}"
+ "fn().x.y = 1;";
// "fn" returns a unescaped local object, we should be able to fold it,
// but we don't currently.
String result = ""
+ "function fn(){var a={x:{}};return a}"
+ "fn().x.y = 1;";
test(options, code, result);
options.computeFunctionSideEffects = true;
test(options, code, result);
}
public void testFoldLocals6() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = ""
+ "function fn(){return {}}"
+ "fn().x.y = 1;";
testSame(options, code);
options.computeFunctionSideEffects = true;
testSame(options, code);
}
public void testFoldLocals7() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = ""
+ "function InternalWidget(){return [];}"
+ "Array.prototype.internalGo = function (){this.x = 2};"
+ "InternalWidget().internalGo();";
testSame(options, code);
options.computeFunctionSideEffects = true;
String optimized = ""
+ "function InternalWidget(){return [];}"
+ "Array.prototype.internalGo = function (){this.x = 2};";
test(options, code, optimized);
}
public void testVarDeclarationsIntoFor() {
CompilerOptions options = createCompilerOptions();
options.collapseVariableDeclarations = false;
String code = "var a = 1; for (var b = 2; ;) {}";
testSame(options, code);
options.collapseVariableDeclarations = false;
test(options, code, "for (var a = 1, b = 2; ;) {}");
}
public void testExploitAssigns() {
CompilerOptions options = createCompilerOptions();
options.collapseVariableDeclarations = false;
String code = "a = 1; b = a; c = b";
testSame(options, code);
options.collapseVariableDeclarations = true;
test(options, code, "c=b=a=1");
}
public void testRecoverOnBadExterns() throws Exception {
// This test is for a bug in a very narrow set of circumstances:
// 1) externs validation has to be off.
// 2) aliasExternals has to be on.
// 3) The user has to reference a "normal" variable in externs.
// This case is handled at checking time by injecting a
// synthetic extern variable, and adding a "@suppress {duplicate}" to
// the normal code at compile time. But optimizations may remove that
// annotation, so we need to make sure that the variable declarations
// are de-duped before that happens.
CompilerOptions options = createCompilerOptions();
options.aliasExternals = true;
externs = ImmutableList.of(
SourceFile.fromCode("externs", "extern.foo"));
test(options,
"var extern; " +
"function f() { return extern + extern + extern + extern; }",
"var extern; " +
"function f() { return extern + extern + extern + extern; }",
VarCheck.UNDEFINED_EXTERN_VAR_ERROR);
}
public void testDuplicateVariablesInExterns() {
CompilerOptions options = createCompilerOptions();
options.checkSymbols = true;
externs = ImmutableList.of(
SourceFile.fromCode("externs",
"var externs = {}; /** @suppress {duplicate} */ var externs = {};"));
testSame(options, "");
}
public void testLanguageMode() {
CompilerOptions options = createCompilerOptions();
options.setLanguageIn(LanguageMode.ECMASCRIPT3);
String code = "var a = {get f(){}}";
Compiler compiler = compile(options, code);
checkUnexpectedErrorsOrWarnings(compiler, 1);
assertEquals(
"JSC_PARSE_ERROR. Parse error. " +
"getters are not supported in older versions of JS. " +
"If you are targeting newer versions of JS, " +
"set the appropriate language_in option. " +
"at i0 line 1 : 0",
compiler.getErrors()[0].toString());
options.setLanguageIn(LanguageMode.ECMASCRIPT5);
testSame(options, code);
options.setLanguageIn(LanguageMode.ECMASCRIPT5_STRICT);
testSame(options, code);
}
public void testLanguageMode2() {
CompilerOptions options = createCompilerOptions();
options.setLanguageIn(LanguageMode.ECMASCRIPT3);
options.setWarningLevel(DiagnosticGroups.ES5_STRICT, CheckLevel.OFF);
String code = "var a = 2; delete a;";
testSame(options, code);
options.setLanguageIn(LanguageMode.ECMASCRIPT5);
testSame(options, code);
options.setLanguageIn(LanguageMode.ECMASCRIPT5_STRICT);
test(options,
code,
code,
StrictModeCheck.DELETE_VARIABLE);
}
public void testIssue598() {
CompilerOptions options = createCompilerOptions();
options.setLanguageIn(LanguageMode.ECMASCRIPT5_STRICT);
WarningLevel.VERBOSE.setOptionsForWarningLevel(options);
options.setLanguageIn(LanguageMode.ECMASCRIPT5);
String code =
"'use strict';\n" +
"function App() {}\n" +
"App.prototype = {\n" +
" get appData() { return this.appData_; },\n" +
" set appData(data) { this.appData_ = data; }\n" +
"};";
Compiler compiler = compile(options, code);
testSame(options, code);
}
public void testIssue701() {
// Check ASCII art in license comments.
String ascii = "/**\n" +
" * @preserve\n" +
" This\n" +
" is\n" +
" ASCII ART\n" +
"*/";
String result = "/*\n\n" +
" This\n" +
" is\n" +
" ASCII ART\n" +
"*/\n";
testSame(createCompilerOptions(), ascii);
assertEquals(result, lastCompiler.toSource());
}
public void testIssue724() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.ADVANCED_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
String code =
"isFunction = function(functionToCheck) {" +
" var getType = {};" +
" return functionToCheck && " +
" getType.toString.apply(functionToCheck) === " +
" '[object Function]';" +
"};";
String result =
"isFunction=function(a){var b={};" +
"return a&&\"[object Function]\"===b.b.a(a)}";
test(options, code, result);
}
public void testIssue730() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.ADVANCED_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
String code =
"/** @constructor */function A() {this.foo = 0; Object.seal(this);}\n" +
"/** @constructor */function B() {this.a = new A();}\n" +
"B.prototype.dostuff = function() {this.a.foo++;alert('hi');}\n" +
"new B().dostuff();\n";
test(options,
code,
"function a(){this.b=0;Object.seal(this)}" +
"(new function(){this.a=new a}).a.b++;" +
"alert(\"hi\")");
options.removeUnusedClassProperties = true;
// This is still a problem when removeUnusedClassProperties are enabled.
test(options,
code,
"function a(){Object.seal(this)}" +
"(new function(){this.a=new a}).a.b++;" +
"alert(\"hi\")");
}
public void testCoaleseVariables() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = false;
options.coalesceVariableNames = true;
String code =
"function f(a) {" +
" if (a) {" +
" return a;" +
" } else {" +
" var b = a;" +
" return b;" +
" }" +
" return a;" +
"}";
String expected =
"function f(a) {" +
" if (a) {" +
" return a;" +
" } else {" +
" a = a;" +
" return a;" +
" }" +
" return a;" +
"}";
test(options, code, expected);
options.foldConstants = true;
options.coalesceVariableNames = false;
code =
"function f(a) {" +
" if (a) {" +
" return a;" +
" } else {" +
" var b = a;" +
" return b;" +
" }" +
" return a;" +
"}";
expected =
"function f(a) {" +
" if (!a) {" +
" var b = a;" +
" return b;" +
" }" +
" return a;" +
"}";
test(options, code, expected);
options.foldConstants = true;
options.coalesceVariableNames = true;
expected =
"function f(a) {" +
" return a;" +
"}";
test(options, code, expected);
}
public void testLateStatementFusion() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
test(options,
"while(a){a();if(b){b();b()}}",
"for(;a;)a(),b&&(b(),b())");
}
public void testLateConstantReordering() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
test(options,
"if (x < 1 || x > 1 || 1 < x || 1 > x) { alert(x) }",
" (1 > x || 1 < x || 1 < x || 1 > x) && alert(x) ");
}
public void testsyntheticBlockOnDeadAssignments() {
CompilerOptions options = createCompilerOptions();
options.deadAssignmentElimination = true;
options.removeUnusedVars = true;
options.syntheticBlockStartMarker = "START";
options.syntheticBlockEndMarker = "END";
test(options, "var x; x = 1; START(); x = 1;END();x()",
"var x; x = 1;{START();{x = 1}END()}x()");
}
public void testBug4152835() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
options.syntheticBlockStartMarker = "START";
options.syntheticBlockEndMarker = "END";
test(options, "START();END()", "{START();{}END()}");
}
public void testBug5786871() {
CompilerOptions options = createCompilerOptions();
options.ideMode = true;
test(options, "function () {}", RhinoErrorReporter.PARSE_ERROR);
}
public void testIssue378() {
CompilerOptions options = createCompilerOptions();
options.inlineVariables = true;
options.flowSensitiveInlineVariables = true;
testSame(options, "function f(c) {var f = c; arguments[0] = this;" +
" f.apply(this, arguments); return this;}");
}
public void testIssue550() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.SIMPLE_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
options.foldConstants = true;
options.inlineVariables = true;
options.flowSensitiveInlineVariables = true;
test(options,
"function f(h) {\n" +
" var a = h;\n" +
" a = a + 'x';\n" +
" a = a + 'y';\n" +
" return a;\n" +
"}",
"function f(a) {return a + 'xy'}");
}
public void testIssue284() {
CompilerOptions options = createCompilerOptions();
options.smartNameRemoval = true;
test(options,
"var goog = {};" +
"goog.inherits = function(x, y) {};" +
"var ns = {};" +
"/** @constructor */" +
"ns.PageSelectionModel = function() {};" +
"/** @constructor */" +
"ns.PageSelectionModel.FooEvent = function() {};" +
"/** @constructor */" +
"ns.PageSelectionModel.SelectEvent = function() {};" +
"goog.inherits(ns.PageSelectionModel.ChangeEvent," +
" ns.PageSelectionModel.FooEvent);",
"");
}
public void testCodingConvention() {
Compiler compiler = new Compiler();
compiler.initOptions(new CompilerOptions());
assertEquals(
compiler.getCodingConvention().getClass().toString(),
ClosureCodingConvention.class.toString());
}
public void testJQueryStringSplitLoops() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
test(options,
"var x=['1','2','3','4','5','6','7']",
"var x='1234567'.split('')");
options = createCompilerOptions();
options.foldConstants = true;
options.computeFunctionSideEffects = false;
options.removeUnusedVars = true;
// If we do splits too early, it would add a side-effect to x.
test(options,
"var x=['1','2','3','4','5','6','7']",
"");
}
public void testAlwaysRunSafetyCheck() {
CompilerOptions options = createCompilerOptions();
options.checkSymbols = false;
options.customPasses = ArrayListMultimap.create();
options.customPasses.put(
CustomPassExecutionTime.BEFORE_OPTIMIZATIONS,
new CompilerPass() {
@Override public void process(Node externs, Node root) {
Node var = root.getLastChild().getFirstChild();
assertEquals(Token.VAR, var.getType());
var.detachFromParent();
}
});
try {
test(options,
"var x = 3; function f() { return x + z; }",
"function f() { return x + z; }");
fail("Expected run-time exception");
} catch (RuntimeException e) {
assertTrue(e.getMessage().indexOf("Unexpected variable x") != -1);
}
}
public void testSuppressEs5StrictWarning() {
CompilerOptions options = createCompilerOptions();
options.setWarningLevel(DiagnosticGroups.ES5_STRICT, CheckLevel.WARNING);
testSame(options,
"/** @suppress{es5Strict} */\n" +
"function f() { var arguments; }");
}
public void testCheckProvidesWarning() {
CompilerOptions options = createCompilerOptions();
options.setWarningLevel(DiagnosticGroups.CHECK_PROVIDES, CheckLevel.WARNING);
options.setCheckProvides(CheckLevel.WARNING);
test(options,
"/** @constructor */\n" +
"function f() { var arguments; }",
DiagnosticType.warning("JSC_MISSING_PROVIDE", "missing goog.provide(''{0}'')"));
}
public void testSuppressCheckProvidesWarning() {
CompilerOptions options = createCompilerOptions();
options.setWarningLevel(DiagnosticGroups.CHECK_PROVIDES, CheckLevel.WARNING);
options.setCheckProvides(CheckLevel.WARNING);
testSame(options,
"/** @constructor\n" +
" * @suppress{checkProvides} */\n" +
"function f() { var arguments; }");
}
public void testRenamePrefixNamespace() {
String code =
"var x = {}; x.FOO = 5; x.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.collapseProperties = true;
options.renamePrefixNamespace = "_";
test(options, code, "_.x$FOO = 5; _.x$bar = 3;");
}
public void testRenamePrefixNamespaceActivatesMoveFunctionDeclarations() {
CompilerOptions options = createCompilerOptions();
String code = "var x = f; function f() { return 3; }";
testSame(options, code);
assertFalse(options.moveFunctionDeclarations);
options.renamePrefixNamespace = "_";
test(options, code, "_.f = function() { return 3; }; _.x = _.f;");
}
public void testBrokenNameSpace() {
CompilerOptions options = createCompilerOptions();
String code = "var goog; goog.provide('i.am.on.a.Horse');" +
"i.am.on.a.Horse = function() {};" +
"i.am.on.a.Horse.prototype.x = function() {};" +
"i.am.on.a.Boat.prototype.y = function() {}";
options.closurePass = true;
options.collapseProperties = true;
options.smartNameRemoval = true;
test(options, code, "");
}
public void testNamelessParameter() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.ADVANCED_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
String code =
"var impl_0;" +
"$load($init());" +
"function $load(){" +
" window['f'] = impl_0;" +
"}" +
"function $init() {" +
" impl_0 = {};" +
"}";
String result =
"window.f = {};";
test(options, code, result);
}
public void testHiddenSideEffect() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.ADVANCED_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
options.setAliasExternals(true);
String code =
"window.offsetWidth;";
String result =
"window.offsetWidth;";
test(options, code, result);
}
public void testNegativeZero() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.ADVANCED_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
test(options,
"function bar(x) { return x; }\n" +
"function foo(x) { print(x / bar(0));\n" +
" print(x / bar(-0)); }\n" +
"foo(3);",
"print(3/0);print(3/-0);");
}
public void testSingletonGetter1() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.ADVANCED_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
options.setCodingConvention(new ClosureCodingConvention());
test(options,
"/** @const */\n" +
"var goog = goog || {};\n" +
"goog.addSingletonGetter = function(ctor) {\n" +
" ctor.getInstance = function() {\n" +
" return ctor.instance_ || (ctor.instance_ = new ctor());\n" +
" };\n" +
"};" +
"function Foo() {}\n" +
"goog.addSingletonGetter(Foo);" +
"Foo.prototype.bar = 1;" +
"function Bar() {}\n" +
"goog.addSingletonGetter(Bar);" +
"Bar.prototype.bar = 1;",
"");
}
public void testIncompleteFunction1() {
CompilerOptions options = createCompilerOptions();
options.ideMode = true;
DiagnosticType[] warnings = new DiagnosticType[]{
RhinoErrorReporter.PARSE_ERROR,
RhinoErrorReporter.PARSE_ERROR};
test(options,
new String[] { "var foo = {bar: function(e) }" },
new String[] { "var foo = {bar: function(e){}};" },
warnings
);
}
public void testIncompleteFunction2() {
CompilerOptions options = createCompilerOptions();
options.ideMode = true;
DiagnosticType[] warnings = new DiagnosticType[]{
RhinoErrorReporter.PARSE_ERROR,
RhinoErrorReporter.PARSE_ERROR,
RhinoErrorReporter.PARSE_ERROR,
RhinoErrorReporter.PARSE_ERROR,
RhinoErrorReporter.PARSE_ERROR,
RhinoErrorReporter.PARSE_ERROR};
test(options,
new String[] { "function hi" },
new String[] { "function hi() {}" },
warnings
);
}
public void testSortingOff() {
CompilerOptions options = new CompilerOptions();
options.closurePass = true;
options.setCodingConvention(new ClosureCodingConvention());
test(options,
new String[] {
"goog.require('goog.beer');",
"goog.provide('goog.beer');"
},
ProcessClosurePrimitives.LATE_PROVIDE_ERROR);
}
public void testUnboundedArrayLiteralInfiniteLoop() {
CompilerOptions options = createCompilerOptions();
options.ideMode = true;
test(options,
"var x = [1, 2",
"var x = [1, 2]",
RhinoErrorReporter.PARSE_ERROR);
}
public void testProvideRequireSameFile() throws Exception {
CompilerOptions options = createCompilerOptions();
options.setDependencyOptions(
new DependencyOptions()
.setDependencySorting(true));
options.closurePass = true;
test(
options,
"goog.provide('x');\ngoog.require('x');",
"var x = {};");
}
public void testDependencySorting() throws Exception {
CompilerOptions options = createCompilerOptions();
options.setDependencyOptions(
new DependencyOptions()
.setDependencySorting(true));
test(
options,
new String[] {
"goog.require('x');",
"goog.provide('x');",
},
new String[] {
"goog.provide('x');",
"goog.require('x');",
// For complicated reasons involving modules,
// the compiler creates a synthetic source file.
"",
});
}
public void testStrictWarningsGuard() throws Exception {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
options.addWarningsGuard(new StrictWarningsGuard());
Compiler compiler = compile(options,
"/** @return {number} */ function f() { return true; }");
assertEquals(1, compiler.getErrors().length);
assertEquals(0, compiler.getWarnings().length);
}
public void testStrictWarningsGuardEmergencyMode() throws Exception {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
options.addWarningsGuard(new StrictWarningsGuard());
options.useEmergencyFailSafe();
Compiler compiler = compile(options,
"/** @return {number} */ function f() { return true; }");
assertEquals(0, compiler.getErrors().length);
assertEquals(1, compiler.getWarnings().length);
}
public void testInlineProperties() {
CompilerOptions options = createCompilerOptions();
CompilationLevel level = CompilationLevel.ADVANCED_OPTIMIZATIONS;
level.setOptionsForCompilationLevel(options);
level.setTypeBasedOptimizationOptions(options);
String code = "" +
"var ns = {};\n" +
"/** @constructor */\n" +
"ns.C = function () {this.someProperty = 1}\n" +
"alert(new ns.C().someProperty + new ns.C().someProperty);\n";
assertTrue(options.inlineProperties);
assertTrue(options.collapseProperties);
// CollapseProperties used to prevent inlining this property.
test(options, code, "alert(2);");
}
public void testCheckConstants1() {
CompilerOptions options = createCompilerOptions();
CompilationLevel level = CompilationLevel.SIMPLE_OPTIMIZATIONS;
level.setOptionsForCompilationLevel(options);
WarningLevel warnings = WarningLevel.QUIET;
warnings.setOptionsForWarningLevel(options);
String code = "" +
"var foo; foo();\n" +
"/** @const */\n" +
"var x = 1; foo(); x = 2;\n";
test(options, code, code);
}
public void testCheckConstants2() {
CompilerOptions options = createCompilerOptions();
CompilationLevel level = CompilationLevel.SIMPLE_OPTIMIZATIONS;
level.setOptionsForCompilationLevel(options);
WarningLevel warnings = WarningLevel.DEFAULT;
warnings.setOptionsForWarningLevel(options);
String code = "" +
"var foo;\n" +
"/** @const */\n" +
"var x = 1; foo(); x = 2;\n";
test(options, code, ConstCheck.CONST_REASSIGNED_VALUE_ERROR);
}
/** Creates a CompilerOptions object with google coding conventions. */
@Override
protected CompilerOptions createCompilerOptions() {
CompilerOptions options = new CompilerOptions();
options.setCodingConvention(new GoogleCodingConvention());
return options;
}
} | // You are a professional Java test case writer, please create a test case named `testDependencySorting` for the issue `Closure-768`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-768
//
// ## Issue-Title:
// Dependency sorting with closurePass set to false no longer works.
//
// ## Issue-Description:
// **What steps will reproduce the problem?**
//
// 1. Instantiate new instance of Compiler
//
// 2. Set closurePass to false to prevent goog.require/goog.provide removal.
// compilerOptions.setClosurePass(false);
//
// 3. Turn dependency sorting on.
// DependencyOptions dependencyOptions = new DependencyOptions();
// dependencyOptions.setDependencySorting(true);
//
// 4. Compile js code
//
//
// What is the expected output?
// Dependent files should be sorted and concatenated in their dependent order.
//
// What do you see instead?
// Dependent files are not sorted.
//
// **What version of the product are you using? On what operating system?**
// > r1824
// mac OS 10.7
//
//
// **Please provide any additional information below.**
// This worked in the r1810 release. However, it looks like this was changed in r1824. The compiler now expects closurePass to be true for dependency sorting to work.
// http://code.google.com/p/closure-compiler/source/detail?path=/trunk/src/com/google/javascript/jscomp/Compiler.java&r=1824
//
// What we are looking for is a way to sort dependencies and concatenate all files in their dependent order without removing the goog.require/goog.provide js calls. Turning closurePass to true causes the goog calls to be replaced. We use this methodology in local development to test our JS code.
//
// Thanks!
//
//
public void testDependencySorting() throws Exception {
| 2,120 | 18 | 2,101 | test/com/google/javascript/jscomp/IntegrationTest.java | test | ```markdown
## Issue-ID: Closure-768
## Issue-Title:
Dependency sorting with closurePass set to false no longer works.
## Issue-Description:
**What steps will reproduce the problem?**
1. Instantiate new instance of Compiler
2. Set closurePass to false to prevent goog.require/goog.provide removal.
compilerOptions.setClosurePass(false);
3. Turn dependency sorting on.
DependencyOptions dependencyOptions = new DependencyOptions();
dependencyOptions.setDependencySorting(true);
4. Compile js code
What is the expected output?
Dependent files should be sorted and concatenated in their dependent order.
What do you see instead?
Dependent files are not sorted.
**What version of the product are you using? On what operating system?**
> r1824
mac OS 10.7
**Please provide any additional information below.**
This worked in the r1810 release. However, it looks like this was changed in r1824. The compiler now expects closurePass to be true for dependency sorting to work.
http://code.google.com/p/closure-compiler/source/detail?path=/trunk/src/com/google/javascript/jscomp/Compiler.java&r=1824
What we are looking for is a way to sort dependencies and concatenate all files in their dependent order without removing the goog.require/goog.provide js calls. Turning closurePass to true causes the goog calls to be replaced. We use this methodology in local development to test our JS code.
Thanks!
```
You are a professional Java test case writer, please create a test case named `testDependencySorting` for the issue `Closure-768`, utilizing the provided issue report information and the following function signature.
```java
public void testDependencySorting() throws Exception {
```
| 2,101 | [
"com.google.javascript.jscomp.Compiler"
] | 8e9ebbd3fd3e375aa54c7191758c747fed591e481ce49384b2b2bf5b0f0d91b0 | public void testDependencySorting() throws Exception | // You are a professional Java test case writer, please create a test case named `testDependencySorting` for the issue `Closure-768`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-768
//
// ## Issue-Title:
// Dependency sorting with closurePass set to false no longer works.
//
// ## Issue-Description:
// **What steps will reproduce the problem?**
//
// 1. Instantiate new instance of Compiler
//
// 2. Set closurePass to false to prevent goog.require/goog.provide removal.
// compilerOptions.setClosurePass(false);
//
// 3. Turn dependency sorting on.
// DependencyOptions dependencyOptions = new DependencyOptions();
// dependencyOptions.setDependencySorting(true);
//
// 4. Compile js code
//
//
// What is the expected output?
// Dependent files should be sorted and concatenated in their dependent order.
//
// What do you see instead?
// Dependent files are not sorted.
//
// **What version of the product are you using? On what operating system?**
// > r1824
// mac OS 10.7
//
//
// **Please provide any additional information below.**
// This worked in the r1810 release. However, it looks like this was changed in r1824. The compiler now expects closurePass to be true for dependency sorting to work.
// http://code.google.com/p/closure-compiler/source/detail?path=/trunk/src/com/google/javascript/jscomp/Compiler.java&r=1824
//
// What we are looking for is a way to sort dependencies and concatenate all files in their dependent order without removing the goog.require/goog.provide js calls. Turning closurePass to true causes the goog calls to be replaced. We use this methodology in local development to test our JS code.
//
// Thanks!
//
//
| Closure | /*
* Copyright 2009 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import com.google.common.collect.ArrayListMultimap;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.Sets;
import com.google.javascript.jscomp.CompilerOptions.LanguageMode;
import com.google.javascript.rhino.Node;
import com.google.javascript.rhino.Token;
/**
* Tests for {@link PassFactory}.
*
* @author [email protected] (Nick Santos)
*/
public class IntegrationTest extends IntegrationTestCase {
private static final String CLOSURE_BOILERPLATE =
"/** @define {boolean} */ var COMPILED = false; var goog = {};" +
"goog.exportSymbol = function() {};";
private static final String CLOSURE_COMPILED =
"var COMPILED = true; var goog$exportSymbol = function() {};";
public void testBug1949424() {
CompilerOptions options = createCompilerOptions();
options.collapseProperties = true;
options.closurePass = true;
test(options, CLOSURE_BOILERPLATE + "goog.provide('FOO'); FOO.bar = 3;",
CLOSURE_COMPILED + "var FOO$bar = 3;");
}
public void testBug1949424_v2() {
CompilerOptions options = createCompilerOptions();
options.collapseProperties = true;
options.closurePass = true;
test(options, CLOSURE_BOILERPLATE + "goog.provide('FOO.BAR'); FOO.BAR = 3;",
CLOSURE_COMPILED + "var FOO$BAR = 3;");
}
public void testBug1956277() {
CompilerOptions options = createCompilerOptions();
options.collapseProperties = true;
options.inlineVariables = true;
test(options, "var CONST = {}; CONST.bar = null;" +
"function f(url) { CONST.bar = url; }",
"var CONST$bar = null; function f(url) { CONST$bar = url; }");
}
public void testBug1962380() {
CompilerOptions options = createCompilerOptions();
options.collapseProperties = true;
options.inlineVariables = true;
options.generateExports = true;
test(options,
CLOSURE_BOILERPLATE + "/** @export */ goog.CONSTANT = 1;" +
"var x = goog.CONSTANT;",
"(function() {})('goog.CONSTANT', 1);" +
"var x = 1;");
}
public void testBug2410122() {
CompilerOptions options = createCompilerOptions();
options.generateExports = true;
options.closurePass = true;
test(options,
"var goog = {};" +
"function F() {}" +
"/** @export */ function G() { goog.base(this); } " +
"goog.inherits(G, F);",
"var goog = {};" +
"function F() {}" +
"function G() { F.call(this); } " +
"goog.inherits(G, F); goog.exportSymbol('G', G);");
}
public void testIssue90() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
options.inlineVariables = true;
options.removeDeadCode = true;
test(options,
"var x; x && alert(1);",
"");
}
public void testClosurePassOff() {
CompilerOptions options = createCompilerOptions();
options.closurePass = false;
testSame(
options,
"var goog = {}; goog.require = function(x) {}; goog.require('foo');");
testSame(
options,
"var goog = {}; goog.getCssName = function(x) {};" +
"goog.getCssName('foo');");
}
public void testClosurePassOn() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
test(
options,
"var goog = {}; goog.require = function(x) {}; goog.require('foo');",
ProcessClosurePrimitives.MISSING_PROVIDE_ERROR);
test(
options,
"/** @define {boolean} */ var COMPILED = false;" +
"var goog = {}; goog.getCssName = function(x) {};" +
"goog.getCssName('foo');",
"var COMPILED = true;" +
"var goog = {}; goog.getCssName = function(x) {};" +
"'foo';");
}
public void testCssNameCheck() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.checkMissingGetCssNameLevel = CheckLevel.ERROR;
options.checkMissingGetCssNameBlacklist = "foo";
test(options, "var x = 'foo';",
CheckMissingGetCssName.MISSING_GETCSSNAME);
}
public void testBug2592659() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.checkTypes = true;
options.checkMissingGetCssNameLevel = CheckLevel.WARNING;
options.checkMissingGetCssNameBlacklist = "foo";
test(options,
"var goog = {};\n" +
"/**\n" +
" * @param {string} className\n" +
" * @param {string=} opt_modifier\n" +
" * @return {string}\n" +
"*/\n" +
"goog.getCssName = function(className, opt_modifier) {}\n" +
"var x = goog.getCssName(123, 'a');",
TypeValidator.TYPE_MISMATCH_WARNING);
}
public void testTypedefBeforeOwner1() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
test(options,
"goog.provide('foo.Bar.Type');\n" +
"goog.provide('foo.Bar');\n" +
"/** @typedef {number} */ foo.Bar.Type;\n" +
"foo.Bar = function() {};",
"var foo = {}; foo.Bar.Type; foo.Bar = function() {};");
}
public void testTypedefBeforeOwner2() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.collapseProperties = true;
test(options,
"goog.provide('foo.Bar.Type');\n" +
"goog.provide('foo.Bar');\n" +
"/** @typedef {number} */ foo.Bar.Type;\n" +
"foo.Bar = function() {};",
"var foo$Bar$Type; var foo$Bar = function() {};");
}
public void testExportedNames() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.variableRenaming = VariableRenamingPolicy.ALL;
test(options,
"/** @define {boolean} */ var COMPILED = false;" +
"var goog = {}; goog.exportSymbol('b', goog);",
"var a = true; var c = {}; c.exportSymbol('b', c);");
test(options,
"/** @define {boolean} */ var COMPILED = false;" +
"var goog = {}; goog.exportSymbol('a', goog);",
"var b = true; var c = {}; c.exportSymbol('a', c);");
}
public void testCheckGlobalThisOn() {
CompilerOptions options = createCompilerOptions();
options.checkSuspiciousCode = true;
options.checkGlobalThisLevel = CheckLevel.ERROR;
test(options, "function f() { this.y = 3; }", CheckGlobalThis.GLOBAL_THIS);
}
public void testSusiciousCodeOff() {
CompilerOptions options = createCompilerOptions();
options.checkSuspiciousCode = false;
options.checkGlobalThisLevel = CheckLevel.ERROR;
test(options, "function f() { this.y = 3; }", CheckGlobalThis.GLOBAL_THIS);
}
public void testCheckGlobalThisOff() {
CompilerOptions options = createCompilerOptions();
options.checkSuspiciousCode = true;
options.checkGlobalThisLevel = CheckLevel.OFF;
testSame(options, "function f() { this.y = 3; }");
}
public void testCheckRequiresAndCheckProvidesOff() {
testSame(createCompilerOptions(), new String[] {
"/** @constructor */ function Foo() {}",
"new Foo();"
});
}
public void testCheckRequiresOn() {
CompilerOptions options = createCompilerOptions();
options.checkRequires = CheckLevel.ERROR;
test(options, new String[] {
"/** @constructor */ function Foo() {}",
"new Foo();"
}, CheckRequiresForConstructors.MISSING_REQUIRE_WARNING);
}
public void testCheckProvidesOn() {
CompilerOptions options = createCompilerOptions();
options.checkProvides = CheckLevel.ERROR;
test(options, new String[] {
"/** @constructor */ function Foo() {}",
"new Foo();"
}, CheckProvides.MISSING_PROVIDE_WARNING);
}
public void testGenerateExportsOff() {
testSame(createCompilerOptions(), "/** @export */ function f() {}");
}
public void testGenerateExportsOn() {
CompilerOptions options = createCompilerOptions();
options.generateExports = true;
test(options, "/** @export */ function f() {}",
"/** @export */ function f() {} goog.exportSymbol('f', f);");
}
public void testExportTestFunctionsOff() {
testSame(createCompilerOptions(), "function testFoo() {}");
}
public void testExportTestFunctionsOn() {
CompilerOptions options = createCompilerOptions();
options.exportTestFunctions = true;
test(options, "function testFoo() {}",
"/** @export */ function testFoo() {}" +
"goog.exportSymbol('testFoo', testFoo);");
}
public void testExpose() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.ADVANCED_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
test(options,
"var x = {eeny: 1, /** @expose */ meeny: 2};" +
"/** @constructor */ var Foo = function() {};" +
"/** @expose */ Foo.prototype.miny = 3;" +
"Foo.prototype.moe = 4;" +
"function moe(a, b) { return a.meeny + b.miny; }" +
"window['x'] = x;" +
"window['Foo'] = Foo;" +
"window['moe'] = moe;",
"function a(){}" +
"a.prototype.miny=3;" +
"window.x={a:1,meeny:2};" +
"window.Foo=a;" +
"window.moe=function(b,c){" +
" return b.meeny+c.miny" +
"}");
}
public void testCheckSymbolsOff() {
CompilerOptions options = createCompilerOptions();
testSame(options, "x = 3;");
}
public void testCheckSymbolsOn() {
CompilerOptions options = createCompilerOptions();
options.checkSymbols = true;
test(options, "x = 3;", VarCheck.UNDEFINED_VAR_ERROR);
}
public void testCheckReferencesOff() {
CompilerOptions options = createCompilerOptions();
testSame(options, "x = 3; var x = 5;");
}
public void testCheckReferencesOn() {
CompilerOptions options = createCompilerOptions();
options.aggressiveVarCheck = CheckLevel.ERROR;
test(options, "x = 3; var x = 5;",
VariableReferenceCheck.UNDECLARED_REFERENCE);
}
public void testInferTypes() {
CompilerOptions options = createCompilerOptions();
options.inferTypes = true;
options.checkTypes = false;
options.closurePass = true;
test(options,
CLOSURE_BOILERPLATE +
"goog.provide('Foo'); /** @enum */ Foo = {a: 3};",
TypeCheck.ENUM_NOT_CONSTANT);
assertTrue(lastCompiler.getErrorManager().getTypedPercent() == 0);
// This does not generate a warning.
test(options, "/** @type {number} */ var n = window.name;",
"var n = window.name;");
assertTrue(lastCompiler.getErrorManager().getTypedPercent() == 0);
}
public void testTypeCheckAndInference() {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
test(options, "/** @type {number} */ var n = window.name;",
TypeValidator.TYPE_MISMATCH_WARNING);
assertTrue(lastCompiler.getErrorManager().getTypedPercent() > 0);
}
public void testTypeNameParser() {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
test(options, "/** @type {n} */ var n = window.name;",
RhinoErrorReporter.TYPE_PARSE_ERROR);
}
// This tests that the TypedScopeCreator is memoized so that it only creates a
// Scope object once for each scope. If, when type inference requests a scope,
// it creates a new one, then multiple JSType objects end up getting created
// for the same local type, and ambiguate will rename the last statement to
// o.a(o.a, o.a), which is bad.
public void testMemoizedTypedScopeCreator() {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
options.ambiguateProperties = true;
options.propertyRenaming = PropertyRenamingPolicy.ALL_UNQUOTED;
test(options, "function someTest() {\n"
+ " /** @constructor */\n"
+ " function Foo() { this.instProp = 3; }\n"
+ " Foo.prototype.protoProp = function(a, b) {};\n"
+ " /** @constructor\n @extends Foo */\n"
+ " function Bar() {}\n"
+ " goog.inherits(Bar, Foo);\n"
+ " var o = new Bar();\n"
+ " o.protoProp(o.protoProp, o.instProp);\n"
+ "}",
"function someTest() {\n"
+ " function Foo() { this.b = 3; }\n"
+ " Foo.prototype.a = function(a, b) {};\n"
+ " function Bar() {}\n"
+ " goog.c(Bar, Foo);\n"
+ " var o = new Bar();\n"
+ " o.a(o.a, o.b);\n"
+ "}");
}
public void testCheckTypes() {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
test(options, "var x = x || {}; x.f = function() {}; x.f(3);",
TypeCheck.WRONG_ARGUMENT_COUNT);
}
public void testReplaceCssNames() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.gatherCssNames = true;
test(options, "/** @define {boolean} */\n"
+ "var COMPILED = false;\n"
+ "goog.setCssNameMapping({'foo':'bar'});\n"
+ "function getCss() {\n"
+ " return goog.getCssName('foo');\n"
+ "}",
"var COMPILED = true;\n"
+ "function getCss() {\n"
+ " return \"bar\";"
+ "}");
assertEquals(
ImmutableMap.of("foo", new Integer(1)),
lastCompiler.getPassConfig().getIntermediateState().cssNames);
}
public void testRemoveClosureAsserts() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
testSame(options,
"var goog = {};"
+ "goog.asserts.assert(goog);");
options.removeClosureAsserts = true;
test(options,
"var goog = {};"
+ "goog.asserts.assert(goog);",
"var goog = {};");
}
public void testDeprecation() {
String code = "/** @deprecated */ function f() { } function g() { f(); }";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.setWarningLevel(DiagnosticGroups.DEPRECATED, CheckLevel.ERROR);
testSame(options, code);
options.checkTypes = true;
test(options, code, CheckAccessControls.DEPRECATED_NAME);
}
public void testVisibility() {
String[] code = {
"/** @private */ function f() { }",
"function g() { f(); }"
};
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.setWarningLevel(DiagnosticGroups.VISIBILITY, CheckLevel.ERROR);
testSame(options, code);
options.checkTypes = true;
test(options, code, CheckAccessControls.BAD_PRIVATE_GLOBAL_ACCESS);
}
public void testUnreachableCode() {
String code = "function f() { return \n 3; }";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.checkUnreachableCode = CheckLevel.ERROR;
test(options, code, CheckUnreachableCode.UNREACHABLE_CODE);
}
public void testMissingReturn() {
String code =
"/** @return {number} */ function f() { if (f) { return 3; } }";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.checkMissingReturn = CheckLevel.ERROR;
testSame(options, code);
options.checkTypes = true;
test(options, code, CheckMissingReturn.MISSING_RETURN_STATEMENT);
}
public void testIdGenerators() {
String code = "function f() {} f('id');";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.idGenerators = Sets.newHashSet("f");
test(options, code, "function f() {} 'a';");
}
public void testOptimizeArgumentsArray() {
String code = "function f() { return arguments[0]; }";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.optimizeArgumentsArray = true;
String argName = "JSCompiler_OptimizeArgumentsArray_p0";
test(options, code,
"function f(" + argName + ") { return " + argName + "; }");
}
public void testOptimizeParameters() {
String code = "function f(a) { return a; } f(true);";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.optimizeParameters = true;
test(options, code, "function f() { var a = true; return a;} f();");
}
public void testOptimizeReturns() {
String code = "function f(a) { return a; } f(true);";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.optimizeReturns = true;
test(options, code, "function f(a) {return;} f(true);");
}
public void testRemoveAbstractMethods() {
String code = CLOSURE_BOILERPLATE +
"var x = {}; x.foo = goog.abstractMethod; x.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.closurePass = true;
options.collapseProperties = true;
test(options, code, CLOSURE_COMPILED + " var x$bar = 3;");
}
public void testCollapseProperties1() {
String code =
"var x = {}; x.FOO = 5; x.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.collapseProperties = true;
test(options, code, "var x$FOO = 5; var x$bar = 3;");
}
public void testCollapseProperties2() {
String code =
"var x = {}; x.FOO = 5; x.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.collapseProperties = true;
options.collapseObjectLiterals = true;
test(options, code, "var x$FOO = 5; var x$bar = 3;");
}
public void testCollapseObjectLiteral1() {
// Verify collapseObjectLiterals does nothing in global scope
String code = "var x = {}; x.FOO = 5; x.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.collapseObjectLiterals = true;
testSame(options, code);
}
public void testCollapseObjectLiteral2() {
String code =
"function f() {var x = {}; x.FOO = 5; x.bar = 3;}";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.collapseObjectLiterals = true;
test(options, code,
"function f(){" +
"var JSCompiler_object_inline_FOO_0;" +
"var JSCompiler_object_inline_bar_1;" +
"JSCompiler_object_inline_FOO_0=5;" +
"JSCompiler_object_inline_bar_1=3}");
}
public void testTightenTypesWithoutTypeCheck() {
CompilerOptions options = createCompilerOptions();
options.tightenTypes = true;
test(options, "", DefaultPassConfig.TIGHTEN_TYPES_WITHOUT_TYPE_CHECK);
}
public void testDisambiguateProperties() {
String code =
"/** @constructor */ function Foo(){} Foo.prototype.bar = 3;" +
"/** @constructor */ function Baz(){} Baz.prototype.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.disambiguateProperties = true;
options.checkTypes = true;
test(options, code,
"function Foo(){} Foo.prototype.Foo_prototype$bar = 3;" +
"function Baz(){} Baz.prototype.Baz_prototype$bar = 3;");
}
public void testMarkPureCalls() {
String testCode = "function foo() {} foo();";
CompilerOptions options = createCompilerOptions();
options.removeDeadCode = true;
testSame(options, testCode);
options.computeFunctionSideEffects = true;
test(options, testCode, "function foo() {}");
}
public void testMarkNoSideEffects() {
String testCode = "noSideEffects();";
CompilerOptions options = createCompilerOptions();
options.removeDeadCode = true;
testSame(options, testCode);
options.markNoSideEffectCalls = true;
test(options, testCode, "");
}
public void testChainedCalls() {
CompilerOptions options = createCompilerOptions();
options.chainCalls = true;
test(
options,
"/** @constructor */ function Foo() {} " +
"Foo.prototype.bar = function() { return this; }; " +
"var f = new Foo();" +
"f.bar(); " +
"f.bar(); ",
"function Foo() {} " +
"Foo.prototype.bar = function() { return this; }; " +
"var f = new Foo();" +
"f.bar().bar();");
}
public void testExtraAnnotationNames() {
CompilerOptions options = createCompilerOptions();
options.setExtraAnnotationNames(Sets.newHashSet("TagA", "TagB"));
test(
options,
"/** @TagA */ var f = new Foo(); /** @TagB */ f.bar();",
"var f = new Foo(); f.bar();");
}
public void testDevirtualizePrototypeMethods() {
CompilerOptions options = createCompilerOptions();
options.devirtualizePrototypeMethods = true;
test(
options,
"/** @constructor */ var Foo = function() {}; " +
"Foo.prototype.bar = function() {};" +
"(new Foo()).bar();",
"var Foo = function() {};" +
"var JSCompiler_StaticMethods_bar = " +
" function(JSCompiler_StaticMethods_bar$self) {};" +
"JSCompiler_StaticMethods_bar(new Foo());");
}
public void testCheckConsts() {
CompilerOptions options = createCompilerOptions();
options.inlineConstantVars = true;
test(options, "var FOO = true; FOO = false",
ConstCheck.CONST_REASSIGNED_VALUE_ERROR);
}
public void testAllChecksOn() {
CompilerOptions options = createCompilerOptions();
options.checkSuspiciousCode = true;
options.checkControlStructures = true;
options.checkRequires = CheckLevel.ERROR;
options.checkProvides = CheckLevel.ERROR;
options.generateExports = true;
options.exportTestFunctions = true;
options.closurePass = true;
options.checkMissingGetCssNameLevel = CheckLevel.ERROR;
options.checkMissingGetCssNameBlacklist = "goog";
options.syntheticBlockStartMarker = "synStart";
options.syntheticBlockEndMarker = "synEnd";
options.checkSymbols = true;
options.aggressiveVarCheck = CheckLevel.ERROR;
options.processObjectPropertyString = true;
options.collapseProperties = true;
test(options, CLOSURE_BOILERPLATE, CLOSURE_COMPILED);
}
public void testTypeCheckingWithSyntheticBlocks() {
CompilerOptions options = createCompilerOptions();
options.syntheticBlockStartMarker = "synStart";
options.syntheticBlockEndMarker = "synEnd";
options.checkTypes = true;
// We used to have a bug where the CFG drew an
// edge straight from synStart to f(progress).
// If that happens, then progress will get type {number|undefined}.
testSame(
options,
"/** @param {number} x */ function f(x) {}" +
"function g() {" +
" synStart('foo');" +
" var progress = 1;" +
" f(progress);" +
" synEnd('foo');" +
"}");
}
public void testCompilerDoesNotBlowUpIfUndefinedSymbols() {
CompilerOptions options = createCompilerOptions();
options.checkSymbols = true;
// Disable the undefined variable check.
options.setWarningLevel(
DiagnosticGroup.forType(VarCheck.UNDEFINED_VAR_ERROR),
CheckLevel.OFF);
// The compiler used to throw an IllegalStateException on this.
testSame(options, "var x = {foo: y};");
}
// Make sure that if we change variables which are constant to have
// $$constant appended to their names, we remove that tag before
// we finish.
public void testConstantTagsMustAlwaysBeRemoved() {
CompilerOptions options = createCompilerOptions();
options.variableRenaming = VariableRenamingPolicy.LOCAL;
String originalText = "var G_GEO_UNKNOWN_ADDRESS=1;\n" +
"function foo() {" +
" var localVar = 2;\n" +
" if (G_GEO_UNKNOWN_ADDRESS == localVar) {\n" +
" alert(\"A\"); }}";
String expectedText = "var G_GEO_UNKNOWN_ADDRESS=1;" +
"function foo(){var a=2;if(G_GEO_UNKNOWN_ADDRESS==a){alert(\"A\")}}";
test(options, originalText, expectedText);
}
public void testClosurePassPreservesJsDoc() {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
options.closurePass = true;
test(options,
CLOSURE_BOILERPLATE +
"goog.provide('Foo'); /** @constructor */ Foo = function() {};" +
"var x = new Foo();",
"var COMPILED=true;var goog={};goog.exportSymbol=function(){};" +
"var Foo=function(){};var x=new Foo");
test(options,
CLOSURE_BOILERPLATE +
"goog.provide('Foo'); /** @enum */ Foo = {a: 3};",
TypeCheck.ENUM_NOT_CONSTANT);
}
public void testProvidedNamespaceIsConst() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.inlineConstantVars = true;
options.collapseProperties = true;
test(options,
"var goog = {}; goog.provide('foo'); " +
"function f() { foo = {};}",
"var foo = {}; function f() { foo = {}; }",
ConstCheck.CONST_REASSIGNED_VALUE_ERROR);
}
public void testProvidedNamespaceIsConst2() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.inlineConstantVars = true;
options.collapseProperties = true;
test(options,
"var goog = {}; goog.provide('foo.bar'); " +
"function f() { foo.bar = {};}",
"var foo$bar = {};" +
"function f() { foo$bar = {}; }",
ConstCheck.CONST_REASSIGNED_VALUE_ERROR);
}
public void testProvidedNamespaceIsConst3() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.inlineConstantVars = true;
options.collapseProperties = true;
test(options,
"var goog = {}; " +
"goog.provide('foo.bar'); goog.provide('foo.bar.baz'); " +
"/** @constructor */ foo.bar = function() {};" +
"/** @constructor */ foo.bar.baz = function() {};",
"var foo$bar = function(){};" +
"var foo$bar$baz = function(){};");
}
public void testProvidedNamespaceIsConst4() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.inlineConstantVars = true;
options.collapseProperties = true;
test(options,
"var goog = {}; goog.provide('foo.Bar'); " +
"var foo = {}; foo.Bar = {};",
"var foo = {}; var foo = {}; foo.Bar = {};");
}
public void testProvidedNamespaceIsConst5() {
CompilerOptions options = createCompilerOptions();
options.closurePass = true;
options.inlineConstantVars = true;
options.collapseProperties = true;
test(options,
"var goog = {}; goog.provide('foo.Bar'); " +
"foo = {}; foo.Bar = {};",
"var foo = {}; foo = {}; foo.Bar = {};");
}
public void testProcessDefinesAlwaysOn() {
test(createCompilerOptions(),
"/** @define {boolean} */ var HI = true; HI = false;",
"var HI = false;false;");
}
public void testProcessDefinesAdditionalReplacements() {
CompilerOptions options = createCompilerOptions();
options.setDefineToBooleanLiteral("HI", false);
test(options,
"/** @define {boolean} */ var HI = true;",
"var HI = false;");
}
public void testReplaceMessages() {
CompilerOptions options = createCompilerOptions();
String prefix = "var goog = {}; goog.getMsg = function() {};";
testSame(options, prefix + "var MSG_HI = goog.getMsg('hi');");
options.messageBundle = new EmptyMessageBundle();
test(options,
prefix + "/** @desc xyz */ var MSG_HI = goog.getMsg('hi');",
prefix + "var MSG_HI = 'hi';");
}
public void testCheckGlobalNames() {
CompilerOptions options = createCompilerOptions();
options.checkGlobalNamesLevel = CheckLevel.ERROR;
test(options, "var x = {}; var y = x.z;",
CheckGlobalNames.UNDEFINED_NAME_WARNING);
}
public void testInlineGetters() {
CompilerOptions options = createCompilerOptions();
String code =
"function Foo() {} Foo.prototype.bar = function() { return 3; };" +
"var x = new Foo(); x.bar();";
testSame(options, code);
options.inlineGetters = true;
test(options, code,
"function Foo() {} Foo.prototype.bar = function() { return 3 };" +
"var x = new Foo(); 3;");
}
public void testInlineGettersWithAmbiguate() {
CompilerOptions options = createCompilerOptions();
String code =
"/** @constructor */" +
"function Foo() {}" +
"/** @type {number} */ Foo.prototype.field;" +
"Foo.prototype.getField = function() { return this.field; };" +
"/** @constructor */" +
"function Bar() {}" +
"/** @type {string} */ Bar.prototype.field;" +
"Bar.prototype.getField = function() { return this.field; };" +
"new Foo().getField();" +
"new Bar().getField();";
testSame(options, code);
options.inlineGetters = true;
test(options, code,
"function Foo() {}" +
"Foo.prototype.field;" +
"Foo.prototype.getField = function() { return this.field; };" +
"function Bar() {}" +
"Bar.prototype.field;" +
"Bar.prototype.getField = function() { return this.field; };" +
"new Foo().field;" +
"new Bar().field;");
options.checkTypes = true;
options.ambiguateProperties = true;
// Propagating the wrong type information may cause ambiguate properties
// to generate bad code.
testSame(options, code);
}
public void testInlineVariables() {
CompilerOptions options = createCompilerOptions();
String code = "function foo() {} var x = 3; foo(x);";
testSame(options, code);
options.inlineVariables = true;
test(options, code, "(function foo() {})(3);");
options.propertyRenaming = PropertyRenamingPolicy.HEURISTIC;
test(options, code, DefaultPassConfig.CANNOT_USE_PROTOTYPE_AND_VAR);
}
public void testInlineConstants() {
CompilerOptions options = createCompilerOptions();
String code = "function foo() {} var x = 3; foo(x); var YYY = 4; foo(YYY);";
testSame(options, code);
options.inlineConstantVars = true;
test(options, code, "function foo() {} var x = 3; foo(x); foo(4);");
}
public void testMinimizeExits() {
CompilerOptions options = createCompilerOptions();
String code =
"function f() {" +
" if (window.foo) return; window.h(); " +
"}";
testSame(options, code);
options.foldConstants = true;
test(
options, code,
"function f() {" +
" window.foo || window.h(); " +
"}");
}
public void testFoldConstants() {
CompilerOptions options = createCompilerOptions();
String code = "if (true) { window.foo(); }";
testSame(options, code);
options.foldConstants = true;
test(options, code, "window.foo();");
}
public void testRemoveUnreachableCode() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { return; f(); }";
testSame(options, code);
options.removeDeadCode = true;
test(options, code, "function f() {}");
}
public void testRemoveUnusedPrototypeProperties1() {
CompilerOptions options = createCompilerOptions();
String code = "function Foo() {} " +
"Foo.prototype.bar = function() { return new Foo(); };";
testSame(options, code);
options.removeUnusedPrototypeProperties = true;
test(options, code, "function Foo() {}");
}
public void testRemoveUnusedPrototypeProperties2() {
CompilerOptions options = createCompilerOptions();
String code = "function Foo() {} " +
"Foo.prototype.bar = function() { return new Foo(); };" +
"function f(x) { x.bar(); }";
testSame(options, code);
options.removeUnusedPrototypeProperties = true;
testSame(options, code);
options.removeUnusedVars = true;
test(options, code, "");
}
public void testSmartNamePass() {
CompilerOptions options = createCompilerOptions();
String code = "function Foo() { this.bar(); } " +
"Foo.prototype.bar = function() { return Foo(); };";
testSame(options, code);
options.smartNameRemoval = true;
test(options, code, "");
}
public void testDeadAssignmentsElimination() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { var x = 3; 4; x = 5; return x; } f(); ";
testSame(options, code);
options.deadAssignmentElimination = true;
testSame(options, code);
options.removeUnusedVars = true;
test(options, code, "function f() { var x = 3; 4; x = 5; return x; } f();");
}
public void testInlineFunctions() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { return 3; } f(); ";
testSame(options, code);
options.inlineFunctions = true;
test(options, code, "3;");
}
public void testRemoveUnusedVars1() {
CompilerOptions options = createCompilerOptions();
String code = "function f(x) {} f();";
testSame(options, code);
options.removeUnusedVars = true;
test(options, code, "function f() {} f();");
}
public void testRemoveUnusedVars2() {
CompilerOptions options = createCompilerOptions();
String code = "(function f(x) {})();var g = function() {}; g();";
testSame(options, code);
options.removeUnusedVars = true;
test(options, code, "(function() {})();var g = function() {}; g();");
options.anonymousFunctionNaming = AnonymousFunctionNamingPolicy.UNMAPPED;
test(options, code, "(function f() {})();var g = function $g$() {}; g();");
}
public void testCrossModuleCodeMotion() {
CompilerOptions options = createCompilerOptions();
String[] code = new String[] {
"var x = 1;",
"x;",
};
testSame(options, code);
options.crossModuleCodeMotion = true;
test(options, code, new String[] {
"",
"var x = 1; x;",
});
}
public void testCrossModuleMethodMotion() {
CompilerOptions options = createCompilerOptions();
String[] code = new String[] {
"var Foo = function() {}; Foo.prototype.bar = function() {};" +
"var x = new Foo();",
"x.bar();",
};
testSame(options, code);
options.crossModuleMethodMotion = true;
test(options, code, new String[] {
CrossModuleMethodMotion.STUB_DECLARATIONS +
"var Foo = function() {};" +
"Foo.prototype.bar=JSCompiler_stubMethod(0); var x=new Foo;",
"Foo.prototype.bar=JSCompiler_unstubMethod(0,function(){}); x.bar()",
});
}
public void testFlowSensitiveInlineVariables1() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { var x = 3; x = 5; return x; }";
testSame(options, code);
options.flowSensitiveInlineVariables = true;
test(options, code, "function f() { var x = 3; return 5; }");
String unusedVar = "function f() { var x; x = 5; return x; } f()";
test(options, unusedVar, "function f() { var x; return 5; } f()");
options.removeUnusedVars = true;
test(options, unusedVar, "function f() { return 5; } f()");
}
public void testFlowSensitiveInlineVariables2() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.SIMPLE_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
test(options,
"function f () {\n" +
" var ab = 0;\n" +
" ab += '-';\n" +
" alert(ab);\n" +
"}",
"function f () {\n" +
" alert('0-');\n" +
"}");
}
public void testCollapseAnonymousFunctions() {
CompilerOptions options = createCompilerOptions();
String code = "var f = function() {};";
testSame(options, code);
options.collapseAnonymousFunctions = true;
test(options, code, "function f() {}");
}
public void testMoveFunctionDeclarations() {
CompilerOptions options = createCompilerOptions();
String code = "var x = f(); function f() { return 3; }";
testSame(options, code);
options.moveFunctionDeclarations = true;
test(options, code, "function f() { return 3; } var x = f();");
}
public void testNameAnonymousFunctions() {
CompilerOptions options = createCompilerOptions();
String code = "var f = function() {};";
testSame(options, code);
options.anonymousFunctionNaming = AnonymousFunctionNamingPolicy.MAPPED;
test(options, code, "var f = function $() {}");
assertNotNull(lastCompiler.getResult().namedAnonFunctionMap);
options.anonymousFunctionNaming = AnonymousFunctionNamingPolicy.UNMAPPED;
test(options, code, "var f = function $f$() {}");
assertNull(lastCompiler.getResult().namedAnonFunctionMap);
}
public void testNameAnonymousFunctionsWithVarRemoval() {
CompilerOptions options = createCompilerOptions();
options.setRemoveUnusedVariables(CompilerOptions.Reach.LOCAL_ONLY);
options.setInlineVariables(true);
String code = "var f = function longName() {}; var g = function() {};" +
"function longerName() {} var i = longerName;";
test(options, code,
"var f = function() {}; var g = function() {}; " +
"var i = function() {};");
options.anonymousFunctionNaming = AnonymousFunctionNamingPolicy.MAPPED;
test(options, code,
"var f = function longName() {}; var g = function $() {};" +
"var i = function longerName(){};");
assertNotNull(lastCompiler.getResult().namedAnonFunctionMap);
options.anonymousFunctionNaming = AnonymousFunctionNamingPolicy.UNMAPPED;
test(options, code,
"var f = function longName() {}; var g = function $g$() {};" +
"var i = function longerName(){};");
assertNull(lastCompiler.getResult().namedAnonFunctionMap);
}
public void testExtractPrototypeMemberDeclarations() {
CompilerOptions options = createCompilerOptions();
String code = "var f = function() {};";
String expected = "var a; var b = function() {}; a = b.prototype;";
for (int i = 0; i < 10; i++) {
code += "f.prototype.a = " + i + ";";
expected += "a.a = " + i + ";";
}
testSame(options, code);
options.extractPrototypeMemberDeclarations = true;
options.variableRenaming = VariableRenamingPolicy.ALL;
test(options, code, expected);
options.propertyRenaming = PropertyRenamingPolicy.HEURISTIC;
options.variableRenaming = VariableRenamingPolicy.OFF;
testSame(options, code);
}
public void testDevirtualizationAndExtractPrototypeMemberDeclarations() {
CompilerOptions options = createCompilerOptions();
options.devirtualizePrototypeMethods = true;
options.collapseAnonymousFunctions = true;
options.extractPrototypeMemberDeclarations = true;
options.variableRenaming = VariableRenamingPolicy.ALL;
String code = "var f = function() {};";
String expected = "var a; function b() {} a = b.prototype;";
for (int i = 0; i < 10; i++) {
code += "f.prototype.argz = function() {arguments};";
code += "f.prototype.devir" + i + " = function() {};";
char letter = (char) ('d' + i);
expected += "a.argz = function() {arguments};";
expected += "function " + letter + "(c){}";
}
code += "var F = new f(); F.argz();";
expected += "var n = new b(); n.argz();";
for (int i = 0; i < 10; i++) {
code += "F.devir" + i + "();";
char letter = (char) ('d' + i);
expected += letter + "(n);";
}
test(options, code, expected);
}
public void testCoalesceVariableNames() {
CompilerOptions options = createCompilerOptions();
String code = "function f() {var x = 3; var y = x; var z = y; return z;}";
testSame(options, code);
options.coalesceVariableNames = true;
test(options, code,
"function f() {var x = 3; x = x; x = x; return x;}");
}
public void testPropertyRenaming() {
CompilerOptions options = createCompilerOptions();
options.propertyAffinity = true;
String code =
"function f() { return this.foo + this['bar'] + this.Baz; }" +
"f.prototype.bar = 3; f.prototype.Baz = 3;";
String heuristic =
"function f() { return this.foo + this['bar'] + this.a; }" +
"f.prototype.bar = 3; f.prototype.a = 3;";
String aggHeuristic =
"function f() { return this.foo + this['b'] + this.a; } " +
"f.prototype.b = 3; f.prototype.a = 3;";
String all =
"function f() { return this.b + this['bar'] + this.a; }" +
"f.prototype.c = 3; f.prototype.a = 3;";
testSame(options, code);
options.propertyRenaming = PropertyRenamingPolicy.HEURISTIC;
test(options, code, heuristic);
options.propertyRenaming = PropertyRenamingPolicy.AGGRESSIVE_HEURISTIC;
test(options, code, aggHeuristic);
options.propertyRenaming = PropertyRenamingPolicy.ALL_UNQUOTED;
test(options, code, all);
}
public void testConvertToDottedProperties() {
CompilerOptions options = createCompilerOptions();
String code =
"function f() { return this['bar']; } f.prototype.bar = 3;";
String expected =
"function f() { return this.bar; } f.prototype.a = 3;";
testSame(options, code);
options.convertToDottedProperties = true;
options.propertyRenaming = PropertyRenamingPolicy.ALL_UNQUOTED;
test(options, code, expected);
}
public void testRewriteFunctionExpressions() {
CompilerOptions options = createCompilerOptions();
String code = "var a = function() {};";
String expected = "function JSCompiler_emptyFn(){return function(){}} " +
"var a = JSCompiler_emptyFn();";
for (int i = 0; i < 10; i++) {
code += "a = function() {};";
expected += "a = JSCompiler_emptyFn();";
}
testSame(options, code);
options.rewriteFunctionExpressions = true;
test(options, code, expected);
}
public void testAliasAllStrings() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { return 'a'; }";
String expected = "var $$S_a = 'a'; function f() { return $$S_a; }";
testSame(options, code);
options.aliasAllStrings = true;
test(options, code, expected);
}
public void testAliasExterns() {
CompilerOptions options = createCompilerOptions();
String code = "function f() { return window + window + window + window; }";
String expected = "var GLOBAL_window = window;" +
"function f() { return GLOBAL_window + GLOBAL_window + " +
" GLOBAL_window + GLOBAL_window; }";
testSame(options, code);
options.aliasExternals = true;
test(options, code, expected);
}
public void testAliasKeywords() {
CompilerOptions options = createCompilerOptions();
String code =
"function f() { return true + true + true + true + true + true; }";
String expected = "var JSCompiler_alias_TRUE = true;" +
"function f() { return JSCompiler_alias_TRUE + " +
" JSCompiler_alias_TRUE + JSCompiler_alias_TRUE + " +
" JSCompiler_alias_TRUE + JSCompiler_alias_TRUE + " +
" JSCompiler_alias_TRUE; }";
testSame(options, code);
options.aliasKeywords = true;
test(options, code, expected);
}
public void testRenameVars1() {
CompilerOptions options = createCompilerOptions();
String code =
"var abc = 3; function f() { var xyz = 5; return abc + xyz; }";
String local = "var abc = 3; function f() { var a = 5; return abc + a; }";
String all = "var a = 3; function c() { var b = 5; return a + b; }";
testSame(options, code);
options.variableRenaming = VariableRenamingPolicy.LOCAL;
test(options, code, local);
options.variableRenaming = VariableRenamingPolicy.ALL;
test(options, code, all);
options.reserveRawExports = true;
}
public void testRenameVars2() {
CompilerOptions options = createCompilerOptions();
options.variableRenaming = VariableRenamingPolicy.ALL;
String code = "var abc = 3; function f() { window['a'] = 5; }";
String noexport = "var a = 3; function b() { window['a'] = 5; }";
String export = "var b = 3; function c() { window['a'] = 5; }";
options.reserveRawExports = false;
test(options, code, noexport);
options.reserveRawExports = true;
test(options, code, export);
}
public void testShadowVaribles() {
CompilerOptions options = createCompilerOptions();
options.variableRenaming = VariableRenamingPolicy.LOCAL;
options.shadowVariables = true;
String code = "var f = function(x) { return function(y) {}}";
String expected = "var f = function(a) { return function(a) {}}";
test(options, code, expected);
}
public void testRenameLabels() {
CompilerOptions options = createCompilerOptions();
String code = "longLabel: while (true) { break longLabel; }";
String expected = "a: while (true) { break a; }";
testSame(options, code);
options.labelRenaming = true;
test(options, code, expected);
}
public void testBadBreakStatementInIdeMode() {
// Ensure that type-checking doesn't crash, even if the CFG is malformed.
// This can happen in IDE mode.
CompilerOptions options = createCompilerOptions();
options.ideMode = true;
options.checkTypes = true;
test(options,
"function f() { try { } catch(e) { break; } }",
RhinoErrorReporter.PARSE_ERROR);
}
public void testIssue63SourceMap() {
CompilerOptions options = createCompilerOptions();
String code = "var a;";
options.skipAllPasses = true;
options.sourceMapOutputPath = "./src.map";
Compiler compiler = compile(options, code);
compiler.toSource();
}
public void testRegExp1() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = "/(a)/.test(\"a\");";
testSame(options, code);
options.computeFunctionSideEffects = true;
String expected = "";
test(options, code, expected);
}
public void testRegExp2() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = "/(a)/.test(\"a\");var a = RegExp.$1";
testSame(options, code);
options.computeFunctionSideEffects = true;
test(options, code, CheckRegExp.REGEXP_REFERENCE);
options.setWarningLevel(DiagnosticGroups.CHECK_REGEXP, CheckLevel.OFF);
testSame(options, code);
}
public void testFoldLocals1() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
// An external object, whose constructor has no side-effects,
// and whose method "go" only modifies the object.
String code = "new Widget().go();";
testSame(options, code);
options.computeFunctionSideEffects = true;
test(options, code, "");
}
public void testFoldLocals2() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
options.checkTypes = true;
// An external function that returns a local object that the
// method "go" that only modifies the object.
String code = "widgetToken().go();";
testSame(options, code);
options.computeFunctionSideEffects = true;
test(options, code, "widgetToken()");
}
public void testFoldLocals3() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
// A function "f" who returns a known local object, and a method that
// modifies only modifies that.
String definition = "function f(){return new Widget()}";
String call = "f().go();";
String code = definition + call;
testSame(options, code);
options.computeFunctionSideEffects = true;
// BROKEN
//test(options, code, definition);
testSame(options, code);
}
public void testFoldLocals4() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = "/** @constructor */\n"
+ "function InternalWidget(){this.x = 1;}"
+ "InternalWidget.prototype.internalGo = function (){this.x = 2};"
+ "new InternalWidget().internalGo();";
testSame(options, code);
options.computeFunctionSideEffects = true;
String optimized = ""
+ "function InternalWidget(){this.x = 1;}"
+ "InternalWidget.prototype.internalGo = function (){this.x = 2};";
test(options, code, optimized);
}
public void testFoldLocals5() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = ""
+ "function fn(){var a={};a.x={};return a}"
+ "fn().x.y = 1;";
// "fn" returns a unescaped local object, we should be able to fold it,
// but we don't currently.
String result = ""
+ "function fn(){var a={x:{}};return a}"
+ "fn().x.y = 1;";
test(options, code, result);
options.computeFunctionSideEffects = true;
test(options, code, result);
}
public void testFoldLocals6() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = ""
+ "function fn(){return {}}"
+ "fn().x.y = 1;";
testSame(options, code);
options.computeFunctionSideEffects = true;
testSame(options, code);
}
public void testFoldLocals7() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
String code = ""
+ "function InternalWidget(){return [];}"
+ "Array.prototype.internalGo = function (){this.x = 2};"
+ "InternalWidget().internalGo();";
testSame(options, code);
options.computeFunctionSideEffects = true;
String optimized = ""
+ "function InternalWidget(){return [];}"
+ "Array.prototype.internalGo = function (){this.x = 2};";
test(options, code, optimized);
}
public void testVarDeclarationsIntoFor() {
CompilerOptions options = createCompilerOptions();
options.collapseVariableDeclarations = false;
String code = "var a = 1; for (var b = 2; ;) {}";
testSame(options, code);
options.collapseVariableDeclarations = false;
test(options, code, "for (var a = 1, b = 2; ;) {}");
}
public void testExploitAssigns() {
CompilerOptions options = createCompilerOptions();
options.collapseVariableDeclarations = false;
String code = "a = 1; b = a; c = b";
testSame(options, code);
options.collapseVariableDeclarations = true;
test(options, code, "c=b=a=1");
}
public void testRecoverOnBadExterns() throws Exception {
// This test is for a bug in a very narrow set of circumstances:
// 1) externs validation has to be off.
// 2) aliasExternals has to be on.
// 3) The user has to reference a "normal" variable in externs.
// This case is handled at checking time by injecting a
// synthetic extern variable, and adding a "@suppress {duplicate}" to
// the normal code at compile time. But optimizations may remove that
// annotation, so we need to make sure that the variable declarations
// are de-duped before that happens.
CompilerOptions options = createCompilerOptions();
options.aliasExternals = true;
externs = ImmutableList.of(
SourceFile.fromCode("externs", "extern.foo"));
test(options,
"var extern; " +
"function f() { return extern + extern + extern + extern; }",
"var extern; " +
"function f() { return extern + extern + extern + extern; }",
VarCheck.UNDEFINED_EXTERN_VAR_ERROR);
}
public void testDuplicateVariablesInExterns() {
CompilerOptions options = createCompilerOptions();
options.checkSymbols = true;
externs = ImmutableList.of(
SourceFile.fromCode("externs",
"var externs = {}; /** @suppress {duplicate} */ var externs = {};"));
testSame(options, "");
}
public void testLanguageMode() {
CompilerOptions options = createCompilerOptions();
options.setLanguageIn(LanguageMode.ECMASCRIPT3);
String code = "var a = {get f(){}}";
Compiler compiler = compile(options, code);
checkUnexpectedErrorsOrWarnings(compiler, 1);
assertEquals(
"JSC_PARSE_ERROR. Parse error. " +
"getters are not supported in older versions of JS. " +
"If you are targeting newer versions of JS, " +
"set the appropriate language_in option. " +
"at i0 line 1 : 0",
compiler.getErrors()[0].toString());
options.setLanguageIn(LanguageMode.ECMASCRIPT5);
testSame(options, code);
options.setLanguageIn(LanguageMode.ECMASCRIPT5_STRICT);
testSame(options, code);
}
public void testLanguageMode2() {
CompilerOptions options = createCompilerOptions();
options.setLanguageIn(LanguageMode.ECMASCRIPT3);
options.setWarningLevel(DiagnosticGroups.ES5_STRICT, CheckLevel.OFF);
String code = "var a = 2; delete a;";
testSame(options, code);
options.setLanguageIn(LanguageMode.ECMASCRIPT5);
testSame(options, code);
options.setLanguageIn(LanguageMode.ECMASCRIPT5_STRICT);
test(options,
code,
code,
StrictModeCheck.DELETE_VARIABLE);
}
public void testIssue598() {
CompilerOptions options = createCompilerOptions();
options.setLanguageIn(LanguageMode.ECMASCRIPT5_STRICT);
WarningLevel.VERBOSE.setOptionsForWarningLevel(options);
options.setLanguageIn(LanguageMode.ECMASCRIPT5);
String code =
"'use strict';\n" +
"function App() {}\n" +
"App.prototype = {\n" +
" get appData() { return this.appData_; },\n" +
" set appData(data) { this.appData_ = data; }\n" +
"};";
Compiler compiler = compile(options, code);
testSame(options, code);
}
public void testIssue701() {
// Check ASCII art in license comments.
String ascii = "/**\n" +
" * @preserve\n" +
" This\n" +
" is\n" +
" ASCII ART\n" +
"*/";
String result = "/*\n\n" +
" This\n" +
" is\n" +
" ASCII ART\n" +
"*/\n";
testSame(createCompilerOptions(), ascii);
assertEquals(result, lastCompiler.toSource());
}
public void testIssue724() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.ADVANCED_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
String code =
"isFunction = function(functionToCheck) {" +
" var getType = {};" +
" return functionToCheck && " +
" getType.toString.apply(functionToCheck) === " +
" '[object Function]';" +
"};";
String result =
"isFunction=function(a){var b={};" +
"return a&&\"[object Function]\"===b.b.a(a)}";
test(options, code, result);
}
public void testIssue730() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.ADVANCED_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
String code =
"/** @constructor */function A() {this.foo = 0; Object.seal(this);}\n" +
"/** @constructor */function B() {this.a = new A();}\n" +
"B.prototype.dostuff = function() {this.a.foo++;alert('hi');}\n" +
"new B().dostuff();\n";
test(options,
code,
"function a(){this.b=0;Object.seal(this)}" +
"(new function(){this.a=new a}).a.b++;" +
"alert(\"hi\")");
options.removeUnusedClassProperties = true;
// This is still a problem when removeUnusedClassProperties are enabled.
test(options,
code,
"function a(){Object.seal(this)}" +
"(new function(){this.a=new a}).a.b++;" +
"alert(\"hi\")");
}
public void testCoaleseVariables() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = false;
options.coalesceVariableNames = true;
String code =
"function f(a) {" +
" if (a) {" +
" return a;" +
" } else {" +
" var b = a;" +
" return b;" +
" }" +
" return a;" +
"}";
String expected =
"function f(a) {" +
" if (a) {" +
" return a;" +
" } else {" +
" a = a;" +
" return a;" +
" }" +
" return a;" +
"}";
test(options, code, expected);
options.foldConstants = true;
options.coalesceVariableNames = false;
code =
"function f(a) {" +
" if (a) {" +
" return a;" +
" } else {" +
" var b = a;" +
" return b;" +
" }" +
" return a;" +
"}";
expected =
"function f(a) {" +
" if (!a) {" +
" var b = a;" +
" return b;" +
" }" +
" return a;" +
"}";
test(options, code, expected);
options.foldConstants = true;
options.coalesceVariableNames = true;
expected =
"function f(a) {" +
" return a;" +
"}";
test(options, code, expected);
}
public void testLateStatementFusion() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
test(options,
"while(a){a();if(b){b();b()}}",
"for(;a;)a(),b&&(b(),b())");
}
public void testLateConstantReordering() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
test(options,
"if (x < 1 || x > 1 || 1 < x || 1 > x) { alert(x) }",
" (1 > x || 1 < x || 1 < x || 1 > x) && alert(x) ");
}
public void testsyntheticBlockOnDeadAssignments() {
CompilerOptions options = createCompilerOptions();
options.deadAssignmentElimination = true;
options.removeUnusedVars = true;
options.syntheticBlockStartMarker = "START";
options.syntheticBlockEndMarker = "END";
test(options, "var x; x = 1; START(); x = 1;END();x()",
"var x; x = 1;{START();{x = 1}END()}x()");
}
public void testBug4152835() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
options.syntheticBlockStartMarker = "START";
options.syntheticBlockEndMarker = "END";
test(options, "START();END()", "{START();{}END()}");
}
public void testBug5786871() {
CompilerOptions options = createCompilerOptions();
options.ideMode = true;
test(options, "function () {}", RhinoErrorReporter.PARSE_ERROR);
}
public void testIssue378() {
CompilerOptions options = createCompilerOptions();
options.inlineVariables = true;
options.flowSensitiveInlineVariables = true;
testSame(options, "function f(c) {var f = c; arguments[0] = this;" +
" f.apply(this, arguments); return this;}");
}
public void testIssue550() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.SIMPLE_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
options.foldConstants = true;
options.inlineVariables = true;
options.flowSensitiveInlineVariables = true;
test(options,
"function f(h) {\n" +
" var a = h;\n" +
" a = a + 'x';\n" +
" a = a + 'y';\n" +
" return a;\n" +
"}",
"function f(a) {return a + 'xy'}");
}
public void testIssue284() {
CompilerOptions options = createCompilerOptions();
options.smartNameRemoval = true;
test(options,
"var goog = {};" +
"goog.inherits = function(x, y) {};" +
"var ns = {};" +
"/** @constructor */" +
"ns.PageSelectionModel = function() {};" +
"/** @constructor */" +
"ns.PageSelectionModel.FooEvent = function() {};" +
"/** @constructor */" +
"ns.PageSelectionModel.SelectEvent = function() {};" +
"goog.inherits(ns.PageSelectionModel.ChangeEvent," +
" ns.PageSelectionModel.FooEvent);",
"");
}
public void testCodingConvention() {
Compiler compiler = new Compiler();
compiler.initOptions(new CompilerOptions());
assertEquals(
compiler.getCodingConvention().getClass().toString(),
ClosureCodingConvention.class.toString());
}
public void testJQueryStringSplitLoops() {
CompilerOptions options = createCompilerOptions();
options.foldConstants = true;
test(options,
"var x=['1','2','3','4','5','6','7']",
"var x='1234567'.split('')");
options = createCompilerOptions();
options.foldConstants = true;
options.computeFunctionSideEffects = false;
options.removeUnusedVars = true;
// If we do splits too early, it would add a side-effect to x.
test(options,
"var x=['1','2','3','4','5','6','7']",
"");
}
public void testAlwaysRunSafetyCheck() {
CompilerOptions options = createCompilerOptions();
options.checkSymbols = false;
options.customPasses = ArrayListMultimap.create();
options.customPasses.put(
CustomPassExecutionTime.BEFORE_OPTIMIZATIONS,
new CompilerPass() {
@Override public void process(Node externs, Node root) {
Node var = root.getLastChild().getFirstChild();
assertEquals(Token.VAR, var.getType());
var.detachFromParent();
}
});
try {
test(options,
"var x = 3; function f() { return x + z; }",
"function f() { return x + z; }");
fail("Expected run-time exception");
} catch (RuntimeException e) {
assertTrue(e.getMessage().indexOf("Unexpected variable x") != -1);
}
}
public void testSuppressEs5StrictWarning() {
CompilerOptions options = createCompilerOptions();
options.setWarningLevel(DiagnosticGroups.ES5_STRICT, CheckLevel.WARNING);
testSame(options,
"/** @suppress{es5Strict} */\n" +
"function f() { var arguments; }");
}
public void testCheckProvidesWarning() {
CompilerOptions options = createCompilerOptions();
options.setWarningLevel(DiagnosticGroups.CHECK_PROVIDES, CheckLevel.WARNING);
options.setCheckProvides(CheckLevel.WARNING);
test(options,
"/** @constructor */\n" +
"function f() { var arguments; }",
DiagnosticType.warning("JSC_MISSING_PROVIDE", "missing goog.provide(''{0}'')"));
}
public void testSuppressCheckProvidesWarning() {
CompilerOptions options = createCompilerOptions();
options.setWarningLevel(DiagnosticGroups.CHECK_PROVIDES, CheckLevel.WARNING);
options.setCheckProvides(CheckLevel.WARNING);
testSame(options,
"/** @constructor\n" +
" * @suppress{checkProvides} */\n" +
"function f() { var arguments; }");
}
public void testRenamePrefixNamespace() {
String code =
"var x = {}; x.FOO = 5; x.bar = 3;";
CompilerOptions options = createCompilerOptions();
testSame(options, code);
options.collapseProperties = true;
options.renamePrefixNamespace = "_";
test(options, code, "_.x$FOO = 5; _.x$bar = 3;");
}
public void testRenamePrefixNamespaceActivatesMoveFunctionDeclarations() {
CompilerOptions options = createCompilerOptions();
String code = "var x = f; function f() { return 3; }";
testSame(options, code);
assertFalse(options.moveFunctionDeclarations);
options.renamePrefixNamespace = "_";
test(options, code, "_.f = function() { return 3; }; _.x = _.f;");
}
public void testBrokenNameSpace() {
CompilerOptions options = createCompilerOptions();
String code = "var goog; goog.provide('i.am.on.a.Horse');" +
"i.am.on.a.Horse = function() {};" +
"i.am.on.a.Horse.prototype.x = function() {};" +
"i.am.on.a.Boat.prototype.y = function() {}";
options.closurePass = true;
options.collapseProperties = true;
options.smartNameRemoval = true;
test(options, code, "");
}
public void testNamelessParameter() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.ADVANCED_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
String code =
"var impl_0;" +
"$load($init());" +
"function $load(){" +
" window['f'] = impl_0;" +
"}" +
"function $init() {" +
" impl_0 = {};" +
"}";
String result =
"window.f = {};";
test(options, code, result);
}
public void testHiddenSideEffect() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.ADVANCED_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
options.setAliasExternals(true);
String code =
"window.offsetWidth;";
String result =
"window.offsetWidth;";
test(options, code, result);
}
public void testNegativeZero() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.ADVANCED_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
test(options,
"function bar(x) { return x; }\n" +
"function foo(x) { print(x / bar(0));\n" +
" print(x / bar(-0)); }\n" +
"foo(3);",
"print(3/0);print(3/-0);");
}
public void testSingletonGetter1() {
CompilerOptions options = createCompilerOptions();
CompilationLevel.ADVANCED_OPTIMIZATIONS
.setOptionsForCompilationLevel(options);
options.setCodingConvention(new ClosureCodingConvention());
test(options,
"/** @const */\n" +
"var goog = goog || {};\n" +
"goog.addSingletonGetter = function(ctor) {\n" +
" ctor.getInstance = function() {\n" +
" return ctor.instance_ || (ctor.instance_ = new ctor());\n" +
" };\n" +
"};" +
"function Foo() {}\n" +
"goog.addSingletonGetter(Foo);" +
"Foo.prototype.bar = 1;" +
"function Bar() {}\n" +
"goog.addSingletonGetter(Bar);" +
"Bar.prototype.bar = 1;",
"");
}
public void testIncompleteFunction1() {
CompilerOptions options = createCompilerOptions();
options.ideMode = true;
DiagnosticType[] warnings = new DiagnosticType[]{
RhinoErrorReporter.PARSE_ERROR,
RhinoErrorReporter.PARSE_ERROR};
test(options,
new String[] { "var foo = {bar: function(e) }" },
new String[] { "var foo = {bar: function(e){}};" },
warnings
);
}
public void testIncompleteFunction2() {
CompilerOptions options = createCompilerOptions();
options.ideMode = true;
DiagnosticType[] warnings = new DiagnosticType[]{
RhinoErrorReporter.PARSE_ERROR,
RhinoErrorReporter.PARSE_ERROR,
RhinoErrorReporter.PARSE_ERROR,
RhinoErrorReporter.PARSE_ERROR,
RhinoErrorReporter.PARSE_ERROR,
RhinoErrorReporter.PARSE_ERROR};
test(options,
new String[] { "function hi" },
new String[] { "function hi() {}" },
warnings
);
}
public void testSortingOff() {
CompilerOptions options = new CompilerOptions();
options.closurePass = true;
options.setCodingConvention(new ClosureCodingConvention());
test(options,
new String[] {
"goog.require('goog.beer');",
"goog.provide('goog.beer');"
},
ProcessClosurePrimitives.LATE_PROVIDE_ERROR);
}
public void testUnboundedArrayLiteralInfiniteLoop() {
CompilerOptions options = createCompilerOptions();
options.ideMode = true;
test(options,
"var x = [1, 2",
"var x = [1, 2]",
RhinoErrorReporter.PARSE_ERROR);
}
public void testProvideRequireSameFile() throws Exception {
CompilerOptions options = createCompilerOptions();
options.setDependencyOptions(
new DependencyOptions()
.setDependencySorting(true));
options.closurePass = true;
test(
options,
"goog.provide('x');\ngoog.require('x');",
"var x = {};");
}
public void testDependencySorting() throws Exception {
CompilerOptions options = createCompilerOptions();
options.setDependencyOptions(
new DependencyOptions()
.setDependencySorting(true));
test(
options,
new String[] {
"goog.require('x');",
"goog.provide('x');",
},
new String[] {
"goog.provide('x');",
"goog.require('x');",
// For complicated reasons involving modules,
// the compiler creates a synthetic source file.
"",
});
}
public void testStrictWarningsGuard() throws Exception {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
options.addWarningsGuard(new StrictWarningsGuard());
Compiler compiler = compile(options,
"/** @return {number} */ function f() { return true; }");
assertEquals(1, compiler.getErrors().length);
assertEquals(0, compiler.getWarnings().length);
}
public void testStrictWarningsGuardEmergencyMode() throws Exception {
CompilerOptions options = createCompilerOptions();
options.checkTypes = true;
options.addWarningsGuard(new StrictWarningsGuard());
options.useEmergencyFailSafe();
Compiler compiler = compile(options,
"/** @return {number} */ function f() { return true; }");
assertEquals(0, compiler.getErrors().length);
assertEquals(1, compiler.getWarnings().length);
}
public void testInlineProperties() {
CompilerOptions options = createCompilerOptions();
CompilationLevel level = CompilationLevel.ADVANCED_OPTIMIZATIONS;
level.setOptionsForCompilationLevel(options);
level.setTypeBasedOptimizationOptions(options);
String code = "" +
"var ns = {};\n" +
"/** @constructor */\n" +
"ns.C = function () {this.someProperty = 1}\n" +
"alert(new ns.C().someProperty + new ns.C().someProperty);\n";
assertTrue(options.inlineProperties);
assertTrue(options.collapseProperties);
// CollapseProperties used to prevent inlining this property.
test(options, code, "alert(2);");
}
public void testCheckConstants1() {
CompilerOptions options = createCompilerOptions();
CompilationLevel level = CompilationLevel.SIMPLE_OPTIMIZATIONS;
level.setOptionsForCompilationLevel(options);
WarningLevel warnings = WarningLevel.QUIET;
warnings.setOptionsForWarningLevel(options);
String code = "" +
"var foo; foo();\n" +
"/** @const */\n" +
"var x = 1; foo(); x = 2;\n";
test(options, code, code);
}
public void testCheckConstants2() {
CompilerOptions options = createCompilerOptions();
CompilationLevel level = CompilationLevel.SIMPLE_OPTIMIZATIONS;
level.setOptionsForCompilationLevel(options);
WarningLevel warnings = WarningLevel.DEFAULT;
warnings.setOptionsForWarningLevel(options);
String code = "" +
"var foo;\n" +
"/** @const */\n" +
"var x = 1; foo(); x = 2;\n";
test(options, code, ConstCheck.CONST_REASSIGNED_VALUE_ERROR);
}
/** Creates a CompilerOptions object with google coding conventions. */
@Override
protected CompilerOptions createCompilerOptions() {
CompilerOptions options = new CompilerOptions();
options.setCodingConvention(new GoogleCodingConvention());
return options;
}
} |
||
public void testRoundLang346() throws Exception
{
TimeZone.setDefault(defaultZone);
dateTimeParser.setTimeZone(defaultZone);
Calendar testCalendar = Calendar.getInstance();
testCalendar.set(2007, 6, 2, 8, 8, 50);
Date date = testCalendar.getTime();
assertEquals("Minute Round Up Failed",
dateTimeParser.parse("July 2, 2007 08:09:00.000"),
DateUtils.round(date, Calendar.MINUTE));
testCalendar.set(2007, 6, 2, 8, 8, 20);
date = testCalendar.getTime();
assertEquals("Minute No Round Failed",
dateTimeParser.parse("July 2, 2007 08:08:00.000"),
DateUtils.round(date, Calendar.MINUTE));
testCalendar.set(2007, 6, 2, 8, 8, 50);
testCalendar.set(Calendar.MILLISECOND, 600);
date = testCalendar.getTime();
assertEquals("Second Round Up with 600 Milli Seconds Failed",
dateTimeParser.parse("July 2, 2007 08:08:51.000"),
DateUtils.round(date, Calendar.SECOND));
testCalendar.set(2007, 6, 2, 8, 8, 50);
testCalendar.set(Calendar.MILLISECOND, 200);
date = testCalendar.getTime();
assertEquals("Second Round Down with 200 Milli Seconds Failed",
dateTimeParser.parse("July 2, 2007 08:08:50.000"),
DateUtils.round(date, Calendar.SECOND));
testCalendar.set(2007, 6, 2, 8, 8, 20);
testCalendar.set(Calendar.MILLISECOND, 600);
date = testCalendar.getTime();
assertEquals("Second Round Up with 200 Milli Seconds Failed",
dateTimeParser.parse("July 2, 2007 08:08:21.000"),
DateUtils.round(date, Calendar.SECOND));
testCalendar.set(2007, 6, 2, 8, 8, 20);
testCalendar.set(Calendar.MILLISECOND, 200);
date = testCalendar.getTime();
assertEquals("Second Round Down with 200 Milli Seconds Failed",
dateTimeParser.parse("July 2, 2007 08:08:20.000"),
DateUtils.round(date, Calendar.SECOND));
testCalendar.set(2007, 6, 2, 8, 8, 50);
date = testCalendar.getTime();
assertEquals("Hour Round Down Failed",
dateTimeParser.parse("July 2, 2007 08:00:00.000"),
DateUtils.round(date, Calendar.HOUR));
testCalendar.set(2007, 6, 2, 8, 31, 50);
date = testCalendar.getTime();
assertEquals("Hour Round Up Failed",
dateTimeParser.parse("July 2, 2007 09:00:00.000"),
DateUtils.round(date, Calendar.HOUR));
} | org.apache.commons.lang.time.DateUtilsTest::testRoundLang346 | src/test/org/apache/commons/lang/time/DateUtilsTest.java | 761 | src/test/org/apache/commons/lang/time/DateUtilsTest.java | testRoundLang346 | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.lang.time;
import java.lang.reflect.Constructor;
import java.lang.reflect.Modifier;
import java.text.DateFormat;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
import java.util.GregorianCalendar;
import java.util.Iterator;
import java.util.Locale;
import java.util.NoSuchElementException;
import java.util.TimeZone;
import junit.framework.AssertionFailedError;
import junit.framework.Test;
import junit.framework.TestCase;
import junit.framework.TestSuite;
import junit.textui.TestRunner;
import org.apache.commons.lang.SystemUtils;
/**
* Unit tests {@link org.apache.commons.lang.time.DateUtils}.
*
* @author <a href="mailto:[email protected]">Serge Knystautas</a>
* @author <a href="mailto:[email protected]">Steven Caswell</a>
*/
public class DateUtilsTest extends TestCase {
private static final long MILLIS_TEST;
static {
GregorianCalendar cal = new GregorianCalendar(2000, 6, 5, 4, 3, 2);
cal.set(Calendar.MILLISECOND, 1);
MILLIS_TEST = cal.getTime().getTime();
}
DateFormat dateParser = null;
DateFormat dateTimeParser = null;
DateFormat timeZoneDateParser = null;
Date dateAmPm1 = null;
Date dateAmPm2 = null;
Date dateAmPm3 = null;
Date dateAmPm4 = null;
Date date0 = null;
Date date1 = null;
Date date2 = null;
Date date3 = null;
Date date4 = null;
Date date5 = null;
Date date6 = null;
Date date7 = null;
Date date8 = null;
Calendar calAmPm1 = null;
Calendar calAmPm2 = null;
Calendar calAmPm3 = null;
Calendar calAmPm4 = null;
Calendar cal1 = null;
Calendar cal2 = null;
Calendar cal3 = null;
Calendar cal4 = null;
Calendar cal5 = null;
Calendar cal6 = null;
Calendar cal7 = null;
Calendar cal8 = null;
TimeZone zone = null;
TimeZone defaultZone = null;
public DateUtilsTest(String name) {
super(name);
}
public static void main(String[] args) {
TestRunner.run(suite());
}
public static Test suite() {
TestSuite suite = new TestSuite(DateUtilsTest.class);
suite.setName("DateUtils Tests");
return suite;
}
protected void setUp() throws Exception {
super.setUp();
dateParser = new SimpleDateFormat("MMM dd, yyyy", Locale.ENGLISH);
dateTimeParser = new SimpleDateFormat("MMM dd, yyyy H:mm:ss.SSS", Locale.ENGLISH);
dateAmPm1 = dateTimeParser.parse("February 3, 2002 01:10:00.000");
dateAmPm2 = dateTimeParser.parse("February 3, 2002 11:10:00.000");
dateAmPm3 = dateTimeParser.parse("February 3, 2002 13:10:00.000");
dateAmPm4 = dateTimeParser.parse("February 3, 2002 19:10:00.000");
date0 = dateTimeParser.parse("February 3, 2002 12:34:56.789");
date1 = dateTimeParser.parse("February 12, 2002 12:34:56.789");
date2 = dateTimeParser.parse("November 18, 2001 1:23:11.321");
defaultZone = TimeZone.getDefault();
zone = TimeZone.getTimeZone("MET");
TimeZone.setDefault(zone);
dateTimeParser.setTimeZone(zone);
date3 = dateTimeParser.parse("March 30, 2003 05:30:45.000");
date4 = dateTimeParser.parse("March 30, 2003 01:10:00.000");
date5 = dateTimeParser.parse("March 30, 2003 01:40:00.000");
date6 = dateTimeParser.parse("March 30, 2003 02:10:00.000");
date7 = dateTimeParser.parse("March 30, 2003 02:40:00.000");
date8 = dateTimeParser.parse("October 26, 2003 05:30:45.000");
dateTimeParser.setTimeZone(defaultZone);
TimeZone.setDefault(defaultZone);
calAmPm1 = Calendar.getInstance();
calAmPm1.setTime(dateAmPm1);
calAmPm2 = Calendar.getInstance();
calAmPm2.setTime(dateAmPm2);
calAmPm3 = Calendar.getInstance();
calAmPm3.setTime(dateAmPm3);
calAmPm4 = Calendar.getInstance();
calAmPm4.setTime(dateAmPm4);
cal1 = Calendar.getInstance();
cal1.setTime(date1);
cal2 = Calendar.getInstance();
cal2.setTime(date2);
TimeZone.setDefault(zone);
cal3 = Calendar.getInstance();
cal3.setTime(date3);
cal4 = Calendar.getInstance();
cal4.setTime(date4);
cal5 = Calendar.getInstance();
cal5.setTime(date5);
cal6 = Calendar.getInstance();
cal6.setTime(date6);
cal7 = Calendar.getInstance();
cal7.setTime(date7);
cal8 = Calendar.getInstance();
cal8.setTime(date8);
TimeZone.setDefault(defaultZone);
}
protected void tearDown() throws Exception {
super.tearDown();
}
//-----------------------------------------------------------------------
public void testConstructor() {
assertNotNull(new DateUtils());
Constructor[] cons = DateUtils.class.getDeclaredConstructors();
assertEquals(1, cons.length);
assertEquals(true, Modifier.isPublic(cons[0].getModifiers()));
assertEquals(true, Modifier.isPublic(DateUtils.class.getModifiers()));
assertEquals(false, Modifier.isFinal(DateUtils.class.getModifiers()));
}
//-----------------------------------------------------------------------
public void testIsSameDay_Date() {
Date date1 = new GregorianCalendar(2004, 6, 9, 13, 45).getTime();
Date date2 = new GregorianCalendar(2004, 6, 9, 13, 45).getTime();
assertEquals(true, DateUtils.isSameDay(date1, date2));
date2 = new GregorianCalendar(2004, 6, 10, 13, 45).getTime();
assertEquals(false, DateUtils.isSameDay(date1, date2));
date1 = new GregorianCalendar(2004, 6, 10, 13, 45).getTime();
assertEquals(true, DateUtils.isSameDay(date1, date2));
date2 = new GregorianCalendar(2005, 6, 10, 13, 45).getTime();
assertEquals(false, DateUtils.isSameDay(date1, date2));
try {
DateUtils.isSameDay((Date) null, (Date) null);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testIsSameDay_Cal() {
GregorianCalendar cal1 = new GregorianCalendar(2004, 6, 9, 13, 45);
GregorianCalendar cal2 = new GregorianCalendar(2004, 6, 9, 13, 45);
assertEquals(true, DateUtils.isSameDay(cal1, cal2));
cal2.add(Calendar.DAY_OF_YEAR, 1);
assertEquals(false, DateUtils.isSameDay(cal1, cal2));
cal1.add(Calendar.DAY_OF_YEAR, 1);
assertEquals(true, DateUtils.isSameDay(cal1, cal2));
cal2.add(Calendar.YEAR, 1);
assertEquals(false, DateUtils.isSameDay(cal1, cal2));
try {
DateUtils.isSameDay((Calendar) null, (Calendar) null);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testIsSameInstant_Date() {
Date date1 = new GregorianCalendar(2004, 6, 9, 13, 45).getTime();
Date date2 = new GregorianCalendar(2004, 6, 9, 13, 45).getTime();
assertEquals(true, DateUtils.isSameInstant(date1, date2));
date2 = new GregorianCalendar(2004, 6, 10, 13, 45).getTime();
assertEquals(false, DateUtils.isSameInstant(date1, date2));
date1 = new GregorianCalendar(2004, 6, 10, 13, 45).getTime();
assertEquals(true, DateUtils.isSameInstant(date1, date2));
date2 = new GregorianCalendar(2005, 6, 10, 13, 45).getTime();
assertEquals(false, DateUtils.isSameInstant(date1, date2));
try {
DateUtils.isSameInstant((Date) null, (Date) null);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testIsSameInstant_Cal() {
GregorianCalendar cal1 = new GregorianCalendar(TimeZone.getTimeZone("GMT+1"));
GregorianCalendar cal2 = new GregorianCalendar(TimeZone.getTimeZone("GMT-1"));
cal1.set(2004, 6, 9, 13, 45, 0);
cal1.set(Calendar.MILLISECOND, 0);
cal2.set(2004, 6, 9, 13, 45, 0);
cal2.set(Calendar.MILLISECOND, 0);
assertEquals(false, DateUtils.isSameInstant(cal1, cal2));
cal2.set(2004, 6, 9, 11, 45, 0);
assertEquals(true, DateUtils.isSameInstant(cal1, cal2));
try {
DateUtils.isSameInstant((Calendar) null, (Calendar) null);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testIsSameLocalTime_Cal() {
GregorianCalendar cal1 = new GregorianCalendar(TimeZone.getTimeZone("GMT+1"));
GregorianCalendar cal2 = new GregorianCalendar(TimeZone.getTimeZone("GMT-1"));
cal1.set(2004, 6, 9, 13, 45, 0);
cal1.set(Calendar.MILLISECOND, 0);
cal2.set(2004, 6, 9, 13, 45, 0);
cal2.set(Calendar.MILLISECOND, 0);
assertEquals(true, DateUtils.isSameLocalTime(cal1, cal2));
cal2.set(2004, 6, 9, 11, 45, 0);
assertEquals(false, DateUtils.isSameLocalTime(cal1, cal2));
try {
DateUtils.isSameLocalTime((Calendar) null, (Calendar) null);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testParseDate() throws Exception {
GregorianCalendar cal = new GregorianCalendar(1972, 11, 3);
String dateStr = "1972-12-03";
String[] parsers = new String[] {"yyyy'-'DDD", "yyyy'-'MM'-'dd", "yyyyMMdd"};
Date date = DateUtils.parseDate(dateStr, parsers);
assertEquals(cal.getTime(), date);
dateStr = "1972-338";
date = DateUtils.parseDate(dateStr, parsers);
assertEquals(cal.getTime(), date);
dateStr = "19721203";
date = DateUtils.parseDate(dateStr, parsers);
assertEquals(cal.getTime(), date);
try {
DateUtils.parseDate("PURPLE", parsers);
fail();
} catch (ParseException ex) {}
try {
DateUtils.parseDate("197212AB", parsers);
fail();
} catch (ParseException ex) {}
try {
DateUtils.parseDate(null, parsers);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateUtils.parseDate(dateStr, null);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testAddYears() throws Exception {
Date base = new Date(MILLIS_TEST);
Date result = DateUtils.addYears(base, 0);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 2000, 6, 5, 4, 3, 2, 1);
result = DateUtils.addYears(base, 1);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 2001, 6, 5, 4, 3, 2, 1);
result = DateUtils.addYears(base, -1);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 1999, 6, 5, 4, 3, 2, 1);
}
//-----------------------------------------------------------------------
public void testAddMonths() {}
// Defects4J: flaky method
// public void testAddMonths() throws Exception {
// Date base = new Date(MILLIS_TEST);
// Date result = DateUtils.addMonths(base, 0);
// assertNotSame(base, result);
// assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
// assertDate(result, 2000, 6, 5, 4, 3, 2, 1);
//
// result = DateUtils.addMonths(base, 1);
// assertNotSame(base, result);
// assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
// assertDate(result, 2000, 7, 5, 4, 3, 2, 1);
//
// result = DateUtils.addMonths(base, -1);
// assertNotSame(base, result);
// assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
// assertDate(result, 2000, 5, 5, 4, 3, 2, 1);
// }
//-----------------------------------------------------------------------
public void testAddWeeks() throws Exception {
Date base = new Date(MILLIS_TEST);
Date result = DateUtils.addWeeks(base, 0);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 2000, 6, 5, 4, 3, 2, 1);
result = DateUtils.addWeeks(base, 1);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 2000, 6, 12, 4, 3, 2, 1);
result = DateUtils.addWeeks(base, -1);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1); // july
assertDate(result, 2000, 5, 28, 4, 3, 2, 1); // june
}
//-----------------------------------------------------------------------
public void testAddDays() throws Exception {
Date base = new Date(MILLIS_TEST);
Date result = DateUtils.addDays(base, 0);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 2000, 6, 5, 4, 3, 2, 1);
result = DateUtils.addDays(base, 1);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 2000, 6, 6, 4, 3, 2, 1);
result = DateUtils.addDays(base, -1);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 2000, 6, 4, 4, 3, 2, 1);
}
//-----------------------------------------------------------------------
public void testAddHours() throws Exception {
Date base = new Date(MILLIS_TEST);
Date result = DateUtils.addHours(base, 0);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 2000, 6, 5, 4, 3, 2, 1);
result = DateUtils.addHours(base, 1);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 2000, 6, 5, 5, 3, 2, 1);
result = DateUtils.addHours(base, -1);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 2000, 6, 5, 3, 3, 2, 1);
}
//-----------------------------------------------------------------------
public void testAddMinutes() throws Exception {
Date base = new Date(MILLIS_TEST);
Date result = DateUtils.addMinutes(base, 0);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 2000, 6, 5, 4, 3, 2, 1);
result = DateUtils.addMinutes(base, 1);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 2000, 6, 5, 4, 4, 2, 1);
result = DateUtils.addMinutes(base, -1);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 2000, 6, 5, 4, 2, 2, 1);
}
//-----------------------------------------------------------------------
public void testAddSeconds() throws Exception {
Date base = new Date(MILLIS_TEST);
Date result = DateUtils.addSeconds(base, 0);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 2000, 6, 5, 4, 3, 2, 1);
result = DateUtils.addSeconds(base, 1);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 2000, 6, 5, 4, 3, 3, 1);
result = DateUtils.addSeconds(base, -1);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 2000, 6, 5, 4, 3, 1, 1);
}
//-----------------------------------------------------------------------
public void testAddMilliseconds() {}
// Defects4J: flaky method
// public void testAddMilliseconds() throws Exception {
// Date base = new Date(MILLIS_TEST);
// Date result = DateUtils.addMilliseconds(base, 0);
// assertNotSame(base, result);
// assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
// assertDate(result, 2000, 6, 5, 4, 3, 2, 1);
//
// result = DateUtils.addMilliseconds(base, 1);
// assertNotSame(base, result);
// assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
// assertDate(result, 2000, 6, 5, 4, 3, 2, 2);
//
// result = DateUtils.addMilliseconds(base, -1);
// assertNotSame(base, result);
// assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
// assertDate(result, 2000, 6, 5, 4, 3, 2, 0);
// }
//-----------------------------------------------------------------------
public void testAddByField() {}
// Defects4J: flaky method
// public void testAddByField() throws Exception {
// Date base = new Date(MILLIS_TEST);
// Date result = DateUtils.add(base, Calendar.YEAR, 0);
// assertNotSame(base, result);
// assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
// assertDate(result, 2000, 6, 5, 4, 3, 2, 1);
//
// result = DateUtils.add(base, Calendar.YEAR, 1);
// assertNotSame(base, result);
// assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
// assertDate(result, 2001, 6, 5, 4, 3, 2, 1);
//
// result = DateUtils.add(base, Calendar.YEAR, -1);
// assertNotSame(base, result);
// assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
// assertDate(result, 1999, 6, 5, 4, 3, 2, 1);
// }
//-----------------------------------------------------------------------
private void assertDate(Date date, int year, int month, int day, int hour, int min, int sec, int mil) throws Exception {
GregorianCalendar cal = new GregorianCalendar();
cal.setTime(date);
assertEquals(year, cal.get(Calendar.YEAR));
assertEquals(month, cal.get(Calendar.MONTH));
assertEquals(day, cal.get(Calendar.DAY_OF_MONTH));
assertEquals(hour, cal.get(Calendar.HOUR_OF_DAY));
assertEquals(min, cal.get(Calendar.MINUTE));
assertEquals(sec, cal.get(Calendar.SECOND));
assertEquals(mil, cal.get(Calendar.MILLISECOND));
}
//-----------------------------------------------------------------------
/**
* Tests various values with the round method
*/
public void testRound() throws Exception {
// tests for public static Date round(Date date, int field)
assertEquals("round year-1 failed",
dateParser.parse("January 1, 2002"),
DateUtils.round(date1, Calendar.YEAR));
assertEquals("round year-2 failed",
dateParser.parse("January 1, 2002"),
DateUtils.round(date2, Calendar.YEAR));
assertEquals("round month-1 failed",
dateParser.parse("February 1, 2002"),
DateUtils.round(date1, Calendar.MONTH));
assertEquals("round month-2 failed",
dateParser.parse("December 1, 2001"),
DateUtils.round(date2, Calendar.MONTH));
assertEquals("round semimonth-0 failed",
dateParser.parse("February 1, 2002"),
DateUtils.round(date0, DateUtils.SEMI_MONTH));
assertEquals("round semimonth-1 failed",
dateParser.parse("February 16, 2002"),
DateUtils.round(date1, DateUtils.SEMI_MONTH));
assertEquals("round semimonth-2 failed",
dateParser.parse("November 16, 2001"),
DateUtils.round(date2, DateUtils.SEMI_MONTH));
assertEquals("round date-1 failed",
dateParser.parse("February 13, 2002"),
DateUtils.round(date1, Calendar.DATE));
assertEquals("round date-2 failed",
dateParser.parse("November 18, 2001"),
DateUtils.round(date2, Calendar.DATE));
assertEquals("round hour-1 failed",
dateTimeParser.parse("February 12, 2002 13:00:00.000"),
DateUtils.round(date1, Calendar.HOUR));
assertEquals("round hour-2 failed",
dateTimeParser.parse("November 18, 2001 1:00:00.000"),
DateUtils.round(date2, Calendar.HOUR));
assertEquals("round minute-1 failed",
dateTimeParser.parse("February 12, 2002 12:35:00.000"),
DateUtils.round(date1, Calendar.MINUTE));
assertEquals("round minute-2 failed",
dateTimeParser.parse("November 18, 2001 1:23:00.000"),
DateUtils.round(date2, Calendar.MINUTE));
assertEquals("round second-1 failed",
dateTimeParser.parse("February 12, 2002 12:34:57.000"),
DateUtils.round(date1, Calendar.SECOND));
assertEquals("round second-2 failed",
dateTimeParser.parse("November 18, 2001 1:23:11.000"),
DateUtils.round(date2, Calendar.SECOND));
assertEquals("truncate ampm-1 failed",
dateTimeParser.parse("February 3, 2002 00:00:00.000"),
DateUtils.round(dateAmPm1, Calendar.AM_PM));
assertEquals("truncate ampm-2 failed",
dateTimeParser.parse("February 4, 2002 00:00:00.000"),
DateUtils.round(dateAmPm2, Calendar.AM_PM));
assertEquals("truncate ampm-3 failed",
dateTimeParser.parse("February 3, 2002 12:00:00.000"),
DateUtils.round(dateAmPm3, Calendar.AM_PM));
assertEquals("truncate ampm-4 failed",
dateTimeParser.parse("February 4, 2002 12:00:00.000"),
DateUtils.round(dateAmPm4, Calendar.AM_PM));
// tests for public static Date round(Object date, int field)
assertEquals("round year-1 failed",
dateParser.parse("January 1, 2002"),
DateUtils.round((Object) date1, Calendar.YEAR));
assertEquals("round year-2 failed",
dateParser.parse("January 1, 2002"),
DateUtils.round((Object) date2, Calendar.YEAR));
assertEquals("round month-1 failed",
dateParser.parse("February 1, 2002"),
DateUtils.round((Object) date1, Calendar.MONTH));
assertEquals("round month-2 failed",
dateParser.parse("December 1, 2001"),
DateUtils.round((Object) date2, Calendar.MONTH));
assertEquals("round semimonth-1 failed",
dateParser.parse("February 16, 2002"),
DateUtils.round((Object) date1, DateUtils.SEMI_MONTH));
assertEquals("round semimonth-2 failed",
dateParser.parse("November 16, 2001"),
DateUtils.round((Object) date2, DateUtils.SEMI_MONTH));
assertEquals("round date-1 failed",
dateParser.parse("February 13, 2002"),
DateUtils.round((Object) date1, Calendar.DATE));
assertEquals("round date-2 failed",
dateParser.parse("November 18, 2001"),
DateUtils.round((Object) date2, Calendar.DATE));
assertEquals("round hour-1 failed",
dateTimeParser.parse("February 12, 2002 13:00:00.000"),
DateUtils.round((Object) date1, Calendar.HOUR));
assertEquals("round hour-2 failed",
dateTimeParser.parse("November 18, 2001 1:00:00.000"),
DateUtils.round((Object) date2, Calendar.HOUR));
assertEquals("round minute-1 failed",
dateTimeParser.parse("February 12, 2002 12:35:00.000"),
DateUtils.round((Object) date1, Calendar.MINUTE));
assertEquals("round minute-2 failed",
dateTimeParser.parse("November 18, 2001 1:23:00.000"),
DateUtils.round((Object) date2, Calendar.MINUTE));
assertEquals("round second-1 failed",
dateTimeParser.parse("February 12, 2002 12:34:57.000"),
DateUtils.round((Object) date1, Calendar.SECOND));
assertEquals("round second-2 failed",
dateTimeParser.parse("November 18, 2001 1:23:11.000"),
DateUtils.round((Object) date2, Calendar.SECOND));
assertEquals("round calendar second-1 failed",
dateTimeParser.parse("February 12, 2002 12:34:57.000"),
DateUtils.round((Object) cal1, Calendar.SECOND));
assertEquals("round calendar second-2 failed",
dateTimeParser.parse("November 18, 2001 1:23:11.000"),
DateUtils.round((Object) cal2, Calendar.SECOND));
assertEquals("truncate ampm-1 failed",
dateTimeParser.parse("February 3, 2002 00:00:00.000"),
DateUtils.round((Object) dateAmPm1, Calendar.AM_PM));
assertEquals("truncate ampm-2 failed",
dateTimeParser.parse("February 4, 2002 00:00:00.000"),
DateUtils.round((Object) dateAmPm2, Calendar.AM_PM));
assertEquals("truncate ampm-3 failed",
dateTimeParser.parse("February 3, 2002 12:00:00.000"),
DateUtils.round((Object) dateAmPm3, Calendar.AM_PM));
assertEquals("truncate ampm-4 failed",
dateTimeParser.parse("February 4, 2002 12:00:00.000"),
DateUtils.round((Object) dateAmPm4, Calendar.AM_PM));
try {
DateUtils.round((Date) null, Calendar.SECOND);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateUtils.round((Calendar) null, Calendar.SECOND);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateUtils.round((Object) null, Calendar.SECOND);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateUtils.round("", Calendar.SECOND);
fail();
} catch (ClassCastException ex) {}
try {
DateUtils.round(date1, -9999);
fail();
} catch(IllegalArgumentException ex) {}
assertEquals("truncate ampm-1 failed",
dateTimeParser.parse("February 3, 2002 00:00:00.000"),
DateUtils.round((Object) calAmPm1, Calendar.AM_PM));
assertEquals("truncate ampm-2 failed",
dateTimeParser.parse("February 4, 2002 00:00:00.000"),
DateUtils.round((Object) calAmPm2, Calendar.AM_PM));
assertEquals("truncate ampm-3 failed",
dateTimeParser.parse("February 3, 2002 12:00:00.000"),
DateUtils.round((Object) calAmPm3, Calendar.AM_PM));
assertEquals("truncate ampm-4 failed",
dateTimeParser.parse("February 4, 2002 12:00:00.000"),
DateUtils.round((Object) calAmPm4, Calendar.AM_PM));
// Fix for http://issues.apache.org/bugzilla/show_bug.cgi?id=25560
// Test rounding across the beginning of daylight saving time
TimeZone.setDefault(zone);
dateTimeParser.setTimeZone(zone);
assertEquals("round MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 00:00:00.000"),
DateUtils.round(date4, Calendar.DATE));
assertEquals("round MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 00:00:00.000"),
DateUtils.round((Object) cal4, Calendar.DATE));
assertEquals("round MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 00:00:00.000"),
DateUtils.round(date5, Calendar.DATE));
assertEquals("round MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 00:00:00.000"),
DateUtils.round((Object) cal5, Calendar.DATE));
assertEquals("round MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 00:00:00.000"),
DateUtils.round(date6, Calendar.DATE));
assertEquals("round MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 00:00:00.000"),
DateUtils.round((Object) cal6, Calendar.DATE));
assertEquals("round MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 00:00:00.000"),
DateUtils.round(date7, Calendar.DATE));
assertEquals("round MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 00:00:00.000"),
DateUtils.round((Object) cal7, Calendar.DATE));
assertEquals("round MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 01:00:00.000"),
DateUtils.round(date4, Calendar.HOUR_OF_DAY));
assertEquals("round MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 01:00:00.000"),
DateUtils.round((Object) cal4, Calendar.HOUR_OF_DAY));
if (SystemUtils.isJavaVersionAtLeast(1.4f)) {
assertEquals("round MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 03:00:00.000"),
DateUtils.round(date5, Calendar.HOUR_OF_DAY));
assertEquals("round MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 03:00:00.000"),
DateUtils.round((Object) cal5, Calendar.HOUR_OF_DAY));
assertEquals("round MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 03:00:00.000"),
DateUtils.round(date6, Calendar.HOUR_OF_DAY));
assertEquals("round MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 03:00:00.000"),
DateUtils.round((Object) cal6, Calendar.HOUR_OF_DAY));
assertEquals("round MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 04:00:00.000"),
DateUtils.round(date7, Calendar.HOUR_OF_DAY));
assertEquals("round MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 04:00:00.000"),
DateUtils.round((Object) cal7, Calendar.HOUR_OF_DAY));
} else {
this.warn("WARNING: Some date rounding tests not run since the current version is " + SystemUtils.JAVA_VERSION);
}
TimeZone.setDefault(defaultZone);
dateTimeParser.setTimeZone(defaultZone);
}
/**
* Tests the Changes Made by LANG-346 to the DateUtils.modify() private method invoked
* by DateUtils.round().
*/
public void testRoundLang346() throws Exception
{
TimeZone.setDefault(defaultZone);
dateTimeParser.setTimeZone(defaultZone);
Calendar testCalendar = Calendar.getInstance();
testCalendar.set(2007, 6, 2, 8, 8, 50);
Date date = testCalendar.getTime();
assertEquals("Minute Round Up Failed",
dateTimeParser.parse("July 2, 2007 08:09:00.000"),
DateUtils.round(date, Calendar.MINUTE));
testCalendar.set(2007, 6, 2, 8, 8, 20);
date = testCalendar.getTime();
assertEquals("Minute No Round Failed",
dateTimeParser.parse("July 2, 2007 08:08:00.000"),
DateUtils.round(date, Calendar.MINUTE));
testCalendar.set(2007, 6, 2, 8, 8, 50);
testCalendar.set(Calendar.MILLISECOND, 600);
date = testCalendar.getTime();
assertEquals("Second Round Up with 600 Milli Seconds Failed",
dateTimeParser.parse("July 2, 2007 08:08:51.000"),
DateUtils.round(date, Calendar.SECOND));
testCalendar.set(2007, 6, 2, 8, 8, 50);
testCalendar.set(Calendar.MILLISECOND, 200);
date = testCalendar.getTime();
assertEquals("Second Round Down with 200 Milli Seconds Failed",
dateTimeParser.parse("July 2, 2007 08:08:50.000"),
DateUtils.round(date, Calendar.SECOND));
testCalendar.set(2007, 6, 2, 8, 8, 20);
testCalendar.set(Calendar.MILLISECOND, 600);
date = testCalendar.getTime();
assertEquals("Second Round Up with 200 Milli Seconds Failed",
dateTimeParser.parse("July 2, 2007 08:08:21.000"),
DateUtils.round(date, Calendar.SECOND));
testCalendar.set(2007, 6, 2, 8, 8, 20);
testCalendar.set(Calendar.MILLISECOND, 200);
date = testCalendar.getTime();
assertEquals("Second Round Down with 200 Milli Seconds Failed",
dateTimeParser.parse("July 2, 2007 08:08:20.000"),
DateUtils.round(date, Calendar.SECOND));
testCalendar.set(2007, 6, 2, 8, 8, 50);
date = testCalendar.getTime();
assertEquals("Hour Round Down Failed",
dateTimeParser.parse("July 2, 2007 08:00:00.000"),
DateUtils.round(date, Calendar.HOUR));
testCalendar.set(2007, 6, 2, 8, 31, 50);
date = testCalendar.getTime();
assertEquals("Hour Round Up Failed",
dateTimeParser.parse("July 2, 2007 09:00:00.000"),
DateUtils.round(date, Calendar.HOUR));
}
/**
* Tests various values with the trunc method
*/
public void testTruncate() throws Exception {
// tests public static Date truncate(Date date, int field)
assertEquals("truncate year-1 failed",
dateParser.parse("January 1, 2002"),
DateUtils.truncate(date1, Calendar.YEAR));
assertEquals("truncate year-2 failed",
dateParser.parse("January 1, 2001"),
DateUtils.truncate(date2, Calendar.YEAR));
assertEquals("truncate month-1 failed",
dateParser.parse("February 1, 2002"),
DateUtils.truncate(date1, Calendar.MONTH));
assertEquals("truncate month-2 failed",
dateParser.parse("November 1, 2001"),
DateUtils.truncate(date2, Calendar.MONTH));
assertEquals("truncate semimonth-1 failed",
dateParser.parse("February 1, 2002"),
DateUtils.truncate(date1, DateUtils.SEMI_MONTH));
assertEquals("truncate semimonth-2 failed",
dateParser.parse("November 16, 2001"),
DateUtils.truncate(date2, DateUtils.SEMI_MONTH));
assertEquals("truncate date-1 failed",
dateParser.parse("February 12, 2002"),
DateUtils.truncate(date1, Calendar.DATE));
assertEquals("truncate date-2 failed",
dateParser.parse("November 18, 2001"),
DateUtils.truncate(date2, Calendar.DATE));
assertEquals("truncate hour-1 failed",
dateTimeParser.parse("February 12, 2002 12:00:00.000"),
DateUtils.truncate(date1, Calendar.HOUR));
assertEquals("truncate hour-2 failed",
dateTimeParser.parse("November 18, 2001 1:00:00.000"),
DateUtils.truncate(date2, Calendar.HOUR));
assertEquals("truncate minute-1 failed",
dateTimeParser.parse("February 12, 2002 12:34:00.000"),
DateUtils.truncate(date1, Calendar.MINUTE));
assertEquals("truncate minute-2 failed",
dateTimeParser.parse("November 18, 2001 1:23:00.000"),
DateUtils.truncate(date2, Calendar.MINUTE));
assertEquals("truncate second-1 failed",
dateTimeParser.parse("February 12, 2002 12:34:56.000"),
DateUtils.truncate(date1, Calendar.SECOND));
assertEquals("truncate second-2 failed",
dateTimeParser.parse("November 18, 2001 1:23:11.000"),
DateUtils.truncate(date2, Calendar.SECOND));
assertEquals("truncate ampm-1 failed",
dateTimeParser.parse("February 3, 2002 00:00:00.000"),
DateUtils.truncate(dateAmPm1, Calendar.AM_PM));
assertEquals("truncate ampm-2 failed",
dateTimeParser.parse("February 3, 2002 00:00:00.000"),
DateUtils.truncate(dateAmPm2, Calendar.AM_PM));
assertEquals("truncate ampm-3 failed",
dateTimeParser.parse("February 3, 2002 12:00:00.000"),
DateUtils.truncate(dateAmPm3, Calendar.AM_PM));
assertEquals("truncate ampm-4 failed",
dateTimeParser.parse("February 3, 2002 12:00:00.000"),
DateUtils.truncate(dateAmPm4, Calendar.AM_PM));
// tests public static Date truncate(Object date, int field)
assertEquals("truncate year-1 failed",
dateParser.parse("January 1, 2002"),
DateUtils.truncate((Object) date1, Calendar.YEAR));
assertEquals("truncate year-2 failed",
dateParser.parse("January 1, 2001"),
DateUtils.truncate((Object) date2, Calendar.YEAR));
assertEquals("truncate month-1 failed",
dateParser.parse("February 1, 2002"),
DateUtils.truncate((Object) date1, Calendar.MONTH));
assertEquals("truncate month-2 failed",
dateParser.parse("November 1, 2001"),
DateUtils.truncate((Object) date2, Calendar.MONTH));
assertEquals("truncate semimonth-1 failed",
dateParser.parse("February 1, 2002"),
DateUtils.truncate((Object) date1, DateUtils.SEMI_MONTH));
assertEquals("truncate semimonth-2 failed",
dateParser.parse("November 16, 2001"),
DateUtils.truncate((Object) date2, DateUtils.SEMI_MONTH));
assertEquals("truncate date-1 failed",
dateParser.parse("February 12, 2002"),
DateUtils.truncate((Object) date1, Calendar.DATE));
assertEquals("truncate date-2 failed",
dateParser.parse("November 18, 2001"),
DateUtils.truncate((Object) date2, Calendar.DATE));
assertEquals("truncate hour-1 failed",
dateTimeParser.parse("February 12, 2002 12:00:00.000"),
DateUtils.truncate((Object) date1, Calendar.HOUR));
assertEquals("truncate hour-2 failed",
dateTimeParser.parse("November 18, 2001 1:00:00.000"),
DateUtils.truncate((Object) date2, Calendar.HOUR));
assertEquals("truncate minute-1 failed",
dateTimeParser.parse("February 12, 2002 12:34:00.000"),
DateUtils.truncate((Object) date1, Calendar.MINUTE));
assertEquals("truncate minute-2 failed",
dateTimeParser.parse("November 18, 2001 1:23:00.000"),
DateUtils.truncate((Object) date2, Calendar.MINUTE));
assertEquals("truncate second-1 failed",
dateTimeParser.parse("February 12, 2002 12:34:56.000"),
DateUtils.truncate((Object) date1, Calendar.SECOND));
assertEquals("truncate second-2 failed",
dateTimeParser.parse("November 18, 2001 1:23:11.000"),
DateUtils.truncate((Object) date2, Calendar.SECOND));
assertEquals("truncate ampm-1 failed",
dateTimeParser.parse("February 3, 2002 00:00:00.000"),
DateUtils.truncate((Object) dateAmPm1, Calendar.AM_PM));
assertEquals("truncate ampm-2 failed",
dateTimeParser.parse("February 3, 2002 00:00:00.000"),
DateUtils.truncate((Object) dateAmPm2, Calendar.AM_PM));
assertEquals("truncate ampm-3 failed",
dateTimeParser.parse("February 3, 2002 12:00:00.000"),
DateUtils.truncate((Object) dateAmPm3, Calendar.AM_PM));
assertEquals("truncate ampm-4 failed",
dateTimeParser.parse("February 3, 2002 12:00:00.000"),
DateUtils.truncate((Object) dateAmPm4, Calendar.AM_PM));
assertEquals("truncate calendar second-1 failed",
dateTimeParser.parse("February 12, 2002 12:34:56.000"),
DateUtils.truncate((Object) cal1, Calendar.SECOND));
assertEquals("truncate calendar second-2 failed",
dateTimeParser.parse("November 18, 2001 1:23:11.000"),
DateUtils.truncate((Object) cal2, Calendar.SECOND));
assertEquals("truncate ampm-1 failed",
dateTimeParser.parse("February 3, 2002 00:00:00.000"),
DateUtils.truncate((Object) calAmPm1, Calendar.AM_PM));
assertEquals("truncate ampm-2 failed",
dateTimeParser.parse("February 3, 2002 00:00:00.000"),
DateUtils.truncate((Object) calAmPm2, Calendar.AM_PM));
assertEquals("truncate ampm-3 failed",
dateTimeParser.parse("February 3, 2002 12:00:00.000"),
DateUtils.truncate((Object) calAmPm3, Calendar.AM_PM));
assertEquals("truncate ampm-4 failed",
dateTimeParser.parse("February 3, 2002 12:00:00.000"),
DateUtils.truncate((Object) calAmPm4, Calendar.AM_PM));
try {
DateUtils.truncate((Date) null, Calendar.SECOND);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateUtils.truncate((Calendar) null, Calendar.SECOND);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateUtils.truncate((Object) null, Calendar.SECOND);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateUtils.truncate("", Calendar.SECOND);
fail();
} catch (ClassCastException ex) {}
// Fix for http://issues.apache.org/bugzilla/show_bug.cgi?id=25560
// Test truncate across beginning of daylight saving time
TimeZone.setDefault(zone);
dateTimeParser.setTimeZone(zone);
assertEquals("truncate MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 00:00:00.000"),
DateUtils.truncate(date3, Calendar.DATE));
assertEquals("truncate MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 00:00:00.000"),
DateUtils.truncate((Object) cal3, Calendar.DATE));
// Test truncate across end of daylight saving time
assertEquals("truncate MET date across DST change-over",
dateTimeParser.parse("October 26, 2003 00:00:00.000"),
DateUtils.truncate(date8, Calendar.DATE));
assertEquals("truncate MET date across DST change-over",
dateTimeParser.parse("October 26, 2003 00:00:00.000"),
DateUtils.truncate((Object) cal8, Calendar.DATE));
TimeZone.setDefault(defaultZone);
dateTimeParser.setTimeZone(defaultZone);
// Bug 31395, large dates
Date endOfTime = new Date(Long.MAX_VALUE); // fyi: Sun Aug 17 07:12:55 CET 292278994 -- 807 millis
GregorianCalendar endCal = new GregorianCalendar();
endCal.setTime(endOfTime);
try {
DateUtils.truncate(endCal, Calendar.DATE);
fail();
} catch (ArithmeticException ex) {}
endCal.set(Calendar.YEAR, 280000001);
try {
DateUtils.truncate(endCal, Calendar.DATE);
fail();
} catch (ArithmeticException ex) {}
endCal.set(Calendar.YEAR, 280000000);
Calendar cal = DateUtils.truncate(endCal, Calendar.DATE);
assertEquals(0, cal.get(Calendar.HOUR));
}
/**
* Tests for LANG-59
*
* see http://issues.apache.org/jira/browse/LANG-59
*/
public void testTruncateLang59() throws Exception {
if (!SystemUtils.isJavaVersionAtLeast(1.4f)) {
this.warn("WARNING: Test for LANG-59 not run since the current version is " + SystemUtils.JAVA_VERSION);
return;
}
// Set TimeZone to Mountain Time
TimeZone MST_MDT = TimeZone.getTimeZone("MST7MDT");
TimeZone.setDefault(MST_MDT);
DateFormat format = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss.SSS z");
format.setTimeZone(MST_MDT);
Date oct31_01MDT = new Date(1099206000000L);
Date oct31MDT = new Date(oct31_01MDT.getTime() - 3600000L); // - 1 hour
Date oct31_01_02MDT = new Date(oct31_01MDT.getTime() + 120000L); // + 2 minutes
Date oct31_01_02_03MDT = new Date(oct31_01_02MDT.getTime() + 3000L); // + 3 seconds
Date oct31_01_02_03_04MDT = new Date(oct31_01_02_03MDT.getTime() + 4L); // + 4 milliseconds
assertEquals("Check 00:00:00.000", "2004-10-31 00:00:00.000 MDT", format.format(oct31MDT));
assertEquals("Check 01:00:00.000", "2004-10-31 01:00:00.000 MDT", format.format(oct31_01MDT));
assertEquals("Check 01:02:00.000", "2004-10-31 01:02:00.000 MDT", format.format(oct31_01_02MDT));
assertEquals("Check 01:02:03.000", "2004-10-31 01:02:03.000 MDT", format.format(oct31_01_02_03MDT));
assertEquals("Check 01:02:03.004", "2004-10-31 01:02:03.004 MDT", format.format(oct31_01_02_03_04MDT));
// ------- Demonstrate Problem -------
Calendar gval = Calendar.getInstance();
gval.setTime(new Date(oct31_01MDT.getTime()));
gval.set(Calendar.MINUTE, gval.get(Calendar.MINUTE)); // set minutes to the same value
assertEquals("Demonstrate Problem", gval.getTime().getTime(), oct31_01MDT.getTime() + 3600000L);
// ---------- Test Truncate ----------
assertEquals("Truncate Calendar.MILLISECOND",
oct31_01_02_03_04MDT, DateUtils.truncate(oct31_01_02_03_04MDT, Calendar.MILLISECOND));
assertEquals("Truncate Calendar.SECOND",
oct31_01_02_03MDT, DateUtils.truncate(oct31_01_02_03_04MDT, Calendar.SECOND));
assertEquals("Truncate Calendar.MINUTE",
oct31_01_02MDT, DateUtils.truncate(oct31_01_02_03_04MDT, Calendar.MINUTE));
assertEquals("Truncate Calendar.HOUR_OF_DAY",
oct31_01MDT, DateUtils.truncate(oct31_01_02_03_04MDT, Calendar.HOUR_OF_DAY));
assertEquals("Truncate Calendar.HOUR",
oct31_01MDT, DateUtils.truncate(oct31_01_02_03_04MDT, Calendar.HOUR));
assertEquals("Truncate Calendar.DATE",
oct31MDT, DateUtils.truncate(oct31_01_02_03_04MDT, Calendar.DATE));
// ---------- Test Round (down) ----------
assertEquals("Round Calendar.MILLISECOND",
oct31_01_02_03_04MDT, DateUtils.round(oct31_01_02_03_04MDT, Calendar.MILLISECOND));
assertEquals("Round Calendar.SECOND",
oct31_01_02_03MDT, DateUtils.round(oct31_01_02_03_04MDT, Calendar.SECOND));
assertEquals("Round Calendar.MINUTE",
oct31_01_02MDT, DateUtils.round(oct31_01_02_03_04MDT, Calendar.MINUTE));
assertEquals("Round Calendar.HOUR_OF_DAY",
oct31_01MDT, DateUtils.round(oct31_01_02_03_04MDT, Calendar.HOUR_OF_DAY));
assertEquals("Round Calendar.HOUR",
oct31_01MDT, DateUtils.round(oct31_01_02_03_04MDT, Calendar.HOUR));
assertEquals("Round Calendar.DATE",
oct31MDT, DateUtils.round(oct31_01_02_03_04MDT, Calendar.DATE));
// restore default time zone
TimeZone.setDefault(defaultZone);
}
/**
* Tests the iterator exceptions
*/
public void testIteratorEx() throws Exception {
try {
DateUtils.iterator(Calendar.getInstance(), -9999);
} catch (IllegalArgumentException ex) {}
try {
DateUtils.iterator((Date) null, DateUtils.RANGE_WEEK_CENTER);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateUtils.iterator((Calendar) null, DateUtils.RANGE_WEEK_CENTER);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateUtils.iterator((Object) null, DateUtils.RANGE_WEEK_CENTER);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateUtils.iterator("", DateUtils.RANGE_WEEK_CENTER);
fail();
} catch (ClassCastException ex) {}
}
/**
* Tests the calendar iterator for week ranges
*/
public void testWeekIterator() throws Exception {
Calendar now = Calendar.getInstance();
for (int i = 0; i< 7; i++) {
Calendar today = DateUtils.truncate(now, Calendar.DATE);
Calendar sunday = DateUtils.truncate(now, Calendar.DATE);
sunday.add(Calendar.DATE, 1 - sunday.get(Calendar.DAY_OF_WEEK));
Calendar monday = DateUtils.truncate(now, Calendar.DATE);
if (monday.get(Calendar.DAY_OF_WEEK) == 1) {
//This is sunday... roll back 6 days
monday.add(Calendar.DATE, -6);
} else {
monday.add(Calendar.DATE, 2 - monday.get(Calendar.DAY_OF_WEEK));
}
Calendar centered = DateUtils.truncate(now, Calendar.DATE);
centered.add(Calendar.DATE, -3);
Iterator it = DateUtils.iterator(now, DateUtils.RANGE_WEEK_SUNDAY);
assertWeekIterator(it, sunday);
it = DateUtils.iterator(now, DateUtils.RANGE_WEEK_MONDAY);
assertWeekIterator(it, monday);
it = DateUtils.iterator(now, DateUtils.RANGE_WEEK_RELATIVE);
assertWeekIterator(it, today);
it = DateUtils.iterator(now, DateUtils.RANGE_WEEK_CENTER);
assertWeekIterator(it, centered);
it = DateUtils.iterator((Object) now, DateUtils.RANGE_WEEK_CENTER);
assertWeekIterator(it, centered);
it = DateUtils.iterator((Object) now.getTime(), DateUtils.RANGE_WEEK_CENTER);
assertWeekIterator(it, centered);
try {
it.next();
fail();
} catch (NoSuchElementException ex) {}
it = DateUtils.iterator(now, DateUtils.RANGE_WEEK_CENTER);
it.next();
try {
it.remove();
} catch( UnsupportedOperationException ex) {}
now.add(Calendar.DATE,1);
}
}
/**
* Tests the calendar iterator for month-based ranges
*/
public void testMonthIterator() throws Exception {
Iterator it = DateUtils.iterator(date1, DateUtils.RANGE_MONTH_SUNDAY);
assertWeekIterator(it,
dateParser.parse("January 27, 2002"),
dateParser.parse("March 2, 2002"));
it = DateUtils.iterator(date1, DateUtils.RANGE_MONTH_MONDAY);
assertWeekIterator(it,
dateParser.parse("January 28, 2002"),
dateParser.parse("March 3, 2002"));
it = DateUtils.iterator(date2, DateUtils.RANGE_MONTH_SUNDAY);
assertWeekIterator(it,
dateParser.parse("October 28, 2001"),
dateParser.parse("December 1, 2001"));
it = DateUtils.iterator(date2, DateUtils.RANGE_MONTH_MONDAY);
assertWeekIterator(it,
dateParser.parse("October 29, 2001"),
dateParser.parse("December 2, 2001"));
}
/**
* This checks that this is a 7 element iterator of Calendar objects
* that are dates (no time), and exactly 1 day spaced after each other.
*/
private static void assertWeekIterator(Iterator it, Calendar start) {
Calendar end = (Calendar) start.clone();
end.add(Calendar.DATE, 6);
assertWeekIterator(it, start, end);
}
/**
* Convenience method for when working with Date objects
*/
private static void assertWeekIterator(Iterator it, Date start, Date end) {
Calendar calStart = Calendar.getInstance();
calStart.setTime(start);
Calendar calEnd = Calendar.getInstance();
calEnd.setTime(end);
assertWeekIterator(it, calStart, calEnd);
}
/**
* This checks that this is a 7 divisble iterator of Calendar objects
* that are dates (no time), and exactly 1 day spaced after each other
* (in addition to the proper start and stop dates)
*/
private static void assertWeekIterator(Iterator it, Calendar start, Calendar end) {
Calendar cal = (Calendar) it.next();
assertEquals("", start, cal, 0);
Calendar last = null;
int count = 1;
while (it.hasNext()) {
//Check this is just a date (no time component)
assertEquals("", cal, DateUtils.truncate(cal, Calendar.DATE), 0);
last = cal;
cal = (Calendar) it.next();
count++;
//Check that this is one day more than the last date
last.add(Calendar.DATE, 1);
assertEquals("", last, cal, 0);
}
if (count % 7 != 0) {
throw new AssertionFailedError("There were " + count + " days in this iterator");
}
assertEquals("", end, cal, 0);
}
/**
* Used to check that Calendar objects are close enough
* delta is in milliseconds
*/
private static void assertEquals(String message, Calendar cal1, Calendar cal2, long delta) {
if (Math.abs(cal1.getTime().getTime() - cal2.getTime().getTime()) > delta) {
throw new AssertionFailedError(
message + " expected " + cal1.getTime() + " but got " + cal2.getTime());
}
}
void warn(String msg) {
System.err.println(msg);
}
}
| // You are a professional Java test case writer, please create a test case named `testRoundLang346` for the issue `Lang-LANG-346`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Lang-LANG-346
//
// ## Issue-Title:
// Dates.round() behaves incorrectly for minutes and seconds
//
// ## Issue-Description:
//
// Get unexpected output for rounding by minutes or seconds.
//
//
// public void testRound()
//
// {
//
// Calendar testCalendar = Calendar.getInstance(TimeZone.getTimeZone("GMT"));
//
// testCalendar.set(2007, 6, 2, 8, 9, 50);
//
// Date date = testCalendar.getTime();
//
// System.out.println("Before round() " + date);
//
// System.out.println("After round() " + DateUtils.round(date, Calendar.MINUTE));
//
// }
//
//
// --2.1 produces
//
// Before round() Mon Jul 02 03:09:50 CDT 2007
//
// After round() Mon Jul 02 03:10:00 CDT 2007 – this is what I would expect
//
//
// --2.2 and 2.3 produces
//
// Before round() Mon Jul 02 03:09:50 CDT 2007
//
// After round() Mon Jul 02 03:01:00 CDT 2007 – this appears to be wrong
//
//
//
//
//
public void testRoundLang346() throws Exception {
| 761 | /**
* Tests the Changes Made by LANG-346 to the DateUtils.modify() private method invoked
* by DateUtils.round().
*/ | 53 | 704 | src/test/org/apache/commons/lang/time/DateUtilsTest.java | src/test | ```markdown
## Issue-ID: Lang-LANG-346
## Issue-Title:
Dates.round() behaves incorrectly for minutes and seconds
## Issue-Description:
Get unexpected output for rounding by minutes or seconds.
public void testRound()
{
Calendar testCalendar = Calendar.getInstance(TimeZone.getTimeZone("GMT"));
testCalendar.set(2007, 6, 2, 8, 9, 50);
Date date = testCalendar.getTime();
System.out.println("Before round() " + date);
System.out.println("After round() " + DateUtils.round(date, Calendar.MINUTE));
}
--2.1 produces
Before round() Mon Jul 02 03:09:50 CDT 2007
After round() Mon Jul 02 03:10:00 CDT 2007 – this is what I would expect
--2.2 and 2.3 produces
Before round() Mon Jul 02 03:09:50 CDT 2007
After round() Mon Jul 02 03:01:00 CDT 2007 – this appears to be wrong
```
You are a professional Java test case writer, please create a test case named `testRoundLang346` for the issue `Lang-LANG-346`, utilizing the provided issue report information and the following function signature.
```java
public void testRoundLang346() throws Exception {
```
| 704 | [
"org.apache.commons.lang.time.DateUtils"
] | 8ef098abb2606bf0f50a8ac6df4bdcd703cf437074a79202ffd1ab7bbfd5471e | public void testRoundLang346() throws Exception
| // You are a professional Java test case writer, please create a test case named `testRoundLang346` for the issue `Lang-LANG-346`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Lang-LANG-346
//
// ## Issue-Title:
// Dates.round() behaves incorrectly for minutes and seconds
//
// ## Issue-Description:
//
// Get unexpected output for rounding by minutes or seconds.
//
//
// public void testRound()
//
// {
//
// Calendar testCalendar = Calendar.getInstance(TimeZone.getTimeZone("GMT"));
//
// testCalendar.set(2007, 6, 2, 8, 9, 50);
//
// Date date = testCalendar.getTime();
//
// System.out.println("Before round() " + date);
//
// System.out.println("After round() " + DateUtils.round(date, Calendar.MINUTE));
//
// }
//
//
// --2.1 produces
//
// Before round() Mon Jul 02 03:09:50 CDT 2007
//
// After round() Mon Jul 02 03:10:00 CDT 2007 – this is what I would expect
//
//
// --2.2 and 2.3 produces
//
// Before round() Mon Jul 02 03:09:50 CDT 2007
//
// After round() Mon Jul 02 03:01:00 CDT 2007 – this appears to be wrong
//
//
//
//
//
| Lang | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.lang.time;
import java.lang.reflect.Constructor;
import java.lang.reflect.Modifier;
import java.text.DateFormat;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
import java.util.GregorianCalendar;
import java.util.Iterator;
import java.util.Locale;
import java.util.NoSuchElementException;
import java.util.TimeZone;
import junit.framework.AssertionFailedError;
import junit.framework.Test;
import junit.framework.TestCase;
import junit.framework.TestSuite;
import junit.textui.TestRunner;
import org.apache.commons.lang.SystemUtils;
/**
* Unit tests {@link org.apache.commons.lang.time.DateUtils}.
*
* @author <a href="mailto:[email protected]">Serge Knystautas</a>
* @author <a href="mailto:[email protected]">Steven Caswell</a>
*/
public class DateUtilsTest extends TestCase {
private static final long MILLIS_TEST;
static {
GregorianCalendar cal = new GregorianCalendar(2000, 6, 5, 4, 3, 2);
cal.set(Calendar.MILLISECOND, 1);
MILLIS_TEST = cal.getTime().getTime();
}
DateFormat dateParser = null;
DateFormat dateTimeParser = null;
DateFormat timeZoneDateParser = null;
Date dateAmPm1 = null;
Date dateAmPm2 = null;
Date dateAmPm3 = null;
Date dateAmPm4 = null;
Date date0 = null;
Date date1 = null;
Date date2 = null;
Date date3 = null;
Date date4 = null;
Date date5 = null;
Date date6 = null;
Date date7 = null;
Date date8 = null;
Calendar calAmPm1 = null;
Calendar calAmPm2 = null;
Calendar calAmPm3 = null;
Calendar calAmPm4 = null;
Calendar cal1 = null;
Calendar cal2 = null;
Calendar cal3 = null;
Calendar cal4 = null;
Calendar cal5 = null;
Calendar cal6 = null;
Calendar cal7 = null;
Calendar cal8 = null;
TimeZone zone = null;
TimeZone defaultZone = null;
public DateUtilsTest(String name) {
super(name);
}
public static void main(String[] args) {
TestRunner.run(suite());
}
public static Test suite() {
TestSuite suite = new TestSuite(DateUtilsTest.class);
suite.setName("DateUtils Tests");
return suite;
}
protected void setUp() throws Exception {
super.setUp();
dateParser = new SimpleDateFormat("MMM dd, yyyy", Locale.ENGLISH);
dateTimeParser = new SimpleDateFormat("MMM dd, yyyy H:mm:ss.SSS", Locale.ENGLISH);
dateAmPm1 = dateTimeParser.parse("February 3, 2002 01:10:00.000");
dateAmPm2 = dateTimeParser.parse("February 3, 2002 11:10:00.000");
dateAmPm3 = dateTimeParser.parse("February 3, 2002 13:10:00.000");
dateAmPm4 = dateTimeParser.parse("February 3, 2002 19:10:00.000");
date0 = dateTimeParser.parse("February 3, 2002 12:34:56.789");
date1 = dateTimeParser.parse("February 12, 2002 12:34:56.789");
date2 = dateTimeParser.parse("November 18, 2001 1:23:11.321");
defaultZone = TimeZone.getDefault();
zone = TimeZone.getTimeZone("MET");
TimeZone.setDefault(zone);
dateTimeParser.setTimeZone(zone);
date3 = dateTimeParser.parse("March 30, 2003 05:30:45.000");
date4 = dateTimeParser.parse("March 30, 2003 01:10:00.000");
date5 = dateTimeParser.parse("March 30, 2003 01:40:00.000");
date6 = dateTimeParser.parse("March 30, 2003 02:10:00.000");
date7 = dateTimeParser.parse("March 30, 2003 02:40:00.000");
date8 = dateTimeParser.parse("October 26, 2003 05:30:45.000");
dateTimeParser.setTimeZone(defaultZone);
TimeZone.setDefault(defaultZone);
calAmPm1 = Calendar.getInstance();
calAmPm1.setTime(dateAmPm1);
calAmPm2 = Calendar.getInstance();
calAmPm2.setTime(dateAmPm2);
calAmPm3 = Calendar.getInstance();
calAmPm3.setTime(dateAmPm3);
calAmPm4 = Calendar.getInstance();
calAmPm4.setTime(dateAmPm4);
cal1 = Calendar.getInstance();
cal1.setTime(date1);
cal2 = Calendar.getInstance();
cal2.setTime(date2);
TimeZone.setDefault(zone);
cal3 = Calendar.getInstance();
cal3.setTime(date3);
cal4 = Calendar.getInstance();
cal4.setTime(date4);
cal5 = Calendar.getInstance();
cal5.setTime(date5);
cal6 = Calendar.getInstance();
cal6.setTime(date6);
cal7 = Calendar.getInstance();
cal7.setTime(date7);
cal8 = Calendar.getInstance();
cal8.setTime(date8);
TimeZone.setDefault(defaultZone);
}
protected void tearDown() throws Exception {
super.tearDown();
}
//-----------------------------------------------------------------------
public void testConstructor() {
assertNotNull(new DateUtils());
Constructor[] cons = DateUtils.class.getDeclaredConstructors();
assertEquals(1, cons.length);
assertEquals(true, Modifier.isPublic(cons[0].getModifiers()));
assertEquals(true, Modifier.isPublic(DateUtils.class.getModifiers()));
assertEquals(false, Modifier.isFinal(DateUtils.class.getModifiers()));
}
//-----------------------------------------------------------------------
public void testIsSameDay_Date() {
Date date1 = new GregorianCalendar(2004, 6, 9, 13, 45).getTime();
Date date2 = new GregorianCalendar(2004, 6, 9, 13, 45).getTime();
assertEquals(true, DateUtils.isSameDay(date1, date2));
date2 = new GregorianCalendar(2004, 6, 10, 13, 45).getTime();
assertEquals(false, DateUtils.isSameDay(date1, date2));
date1 = new GregorianCalendar(2004, 6, 10, 13, 45).getTime();
assertEquals(true, DateUtils.isSameDay(date1, date2));
date2 = new GregorianCalendar(2005, 6, 10, 13, 45).getTime();
assertEquals(false, DateUtils.isSameDay(date1, date2));
try {
DateUtils.isSameDay((Date) null, (Date) null);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testIsSameDay_Cal() {
GregorianCalendar cal1 = new GregorianCalendar(2004, 6, 9, 13, 45);
GregorianCalendar cal2 = new GregorianCalendar(2004, 6, 9, 13, 45);
assertEquals(true, DateUtils.isSameDay(cal1, cal2));
cal2.add(Calendar.DAY_OF_YEAR, 1);
assertEquals(false, DateUtils.isSameDay(cal1, cal2));
cal1.add(Calendar.DAY_OF_YEAR, 1);
assertEquals(true, DateUtils.isSameDay(cal1, cal2));
cal2.add(Calendar.YEAR, 1);
assertEquals(false, DateUtils.isSameDay(cal1, cal2));
try {
DateUtils.isSameDay((Calendar) null, (Calendar) null);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testIsSameInstant_Date() {
Date date1 = new GregorianCalendar(2004, 6, 9, 13, 45).getTime();
Date date2 = new GregorianCalendar(2004, 6, 9, 13, 45).getTime();
assertEquals(true, DateUtils.isSameInstant(date1, date2));
date2 = new GregorianCalendar(2004, 6, 10, 13, 45).getTime();
assertEquals(false, DateUtils.isSameInstant(date1, date2));
date1 = new GregorianCalendar(2004, 6, 10, 13, 45).getTime();
assertEquals(true, DateUtils.isSameInstant(date1, date2));
date2 = new GregorianCalendar(2005, 6, 10, 13, 45).getTime();
assertEquals(false, DateUtils.isSameInstant(date1, date2));
try {
DateUtils.isSameInstant((Date) null, (Date) null);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testIsSameInstant_Cal() {
GregorianCalendar cal1 = new GregorianCalendar(TimeZone.getTimeZone("GMT+1"));
GregorianCalendar cal2 = new GregorianCalendar(TimeZone.getTimeZone("GMT-1"));
cal1.set(2004, 6, 9, 13, 45, 0);
cal1.set(Calendar.MILLISECOND, 0);
cal2.set(2004, 6, 9, 13, 45, 0);
cal2.set(Calendar.MILLISECOND, 0);
assertEquals(false, DateUtils.isSameInstant(cal1, cal2));
cal2.set(2004, 6, 9, 11, 45, 0);
assertEquals(true, DateUtils.isSameInstant(cal1, cal2));
try {
DateUtils.isSameInstant((Calendar) null, (Calendar) null);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testIsSameLocalTime_Cal() {
GregorianCalendar cal1 = new GregorianCalendar(TimeZone.getTimeZone("GMT+1"));
GregorianCalendar cal2 = new GregorianCalendar(TimeZone.getTimeZone("GMT-1"));
cal1.set(2004, 6, 9, 13, 45, 0);
cal1.set(Calendar.MILLISECOND, 0);
cal2.set(2004, 6, 9, 13, 45, 0);
cal2.set(Calendar.MILLISECOND, 0);
assertEquals(true, DateUtils.isSameLocalTime(cal1, cal2));
cal2.set(2004, 6, 9, 11, 45, 0);
assertEquals(false, DateUtils.isSameLocalTime(cal1, cal2));
try {
DateUtils.isSameLocalTime((Calendar) null, (Calendar) null);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testParseDate() throws Exception {
GregorianCalendar cal = new GregorianCalendar(1972, 11, 3);
String dateStr = "1972-12-03";
String[] parsers = new String[] {"yyyy'-'DDD", "yyyy'-'MM'-'dd", "yyyyMMdd"};
Date date = DateUtils.parseDate(dateStr, parsers);
assertEquals(cal.getTime(), date);
dateStr = "1972-338";
date = DateUtils.parseDate(dateStr, parsers);
assertEquals(cal.getTime(), date);
dateStr = "19721203";
date = DateUtils.parseDate(dateStr, parsers);
assertEquals(cal.getTime(), date);
try {
DateUtils.parseDate("PURPLE", parsers);
fail();
} catch (ParseException ex) {}
try {
DateUtils.parseDate("197212AB", parsers);
fail();
} catch (ParseException ex) {}
try {
DateUtils.parseDate(null, parsers);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateUtils.parseDate(dateStr, null);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void testAddYears() throws Exception {
Date base = new Date(MILLIS_TEST);
Date result = DateUtils.addYears(base, 0);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 2000, 6, 5, 4, 3, 2, 1);
result = DateUtils.addYears(base, 1);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 2001, 6, 5, 4, 3, 2, 1);
result = DateUtils.addYears(base, -1);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 1999, 6, 5, 4, 3, 2, 1);
}
//-----------------------------------------------------------------------
public void testAddMonths() {}
// Defects4J: flaky method
// public void testAddMonths() throws Exception {
// Date base = new Date(MILLIS_TEST);
// Date result = DateUtils.addMonths(base, 0);
// assertNotSame(base, result);
// assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
// assertDate(result, 2000, 6, 5, 4, 3, 2, 1);
//
// result = DateUtils.addMonths(base, 1);
// assertNotSame(base, result);
// assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
// assertDate(result, 2000, 7, 5, 4, 3, 2, 1);
//
// result = DateUtils.addMonths(base, -1);
// assertNotSame(base, result);
// assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
// assertDate(result, 2000, 5, 5, 4, 3, 2, 1);
// }
//-----------------------------------------------------------------------
public void testAddWeeks() throws Exception {
Date base = new Date(MILLIS_TEST);
Date result = DateUtils.addWeeks(base, 0);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 2000, 6, 5, 4, 3, 2, 1);
result = DateUtils.addWeeks(base, 1);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 2000, 6, 12, 4, 3, 2, 1);
result = DateUtils.addWeeks(base, -1);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1); // july
assertDate(result, 2000, 5, 28, 4, 3, 2, 1); // june
}
//-----------------------------------------------------------------------
public void testAddDays() throws Exception {
Date base = new Date(MILLIS_TEST);
Date result = DateUtils.addDays(base, 0);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 2000, 6, 5, 4, 3, 2, 1);
result = DateUtils.addDays(base, 1);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 2000, 6, 6, 4, 3, 2, 1);
result = DateUtils.addDays(base, -1);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 2000, 6, 4, 4, 3, 2, 1);
}
//-----------------------------------------------------------------------
public void testAddHours() throws Exception {
Date base = new Date(MILLIS_TEST);
Date result = DateUtils.addHours(base, 0);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 2000, 6, 5, 4, 3, 2, 1);
result = DateUtils.addHours(base, 1);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 2000, 6, 5, 5, 3, 2, 1);
result = DateUtils.addHours(base, -1);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 2000, 6, 5, 3, 3, 2, 1);
}
//-----------------------------------------------------------------------
public void testAddMinutes() throws Exception {
Date base = new Date(MILLIS_TEST);
Date result = DateUtils.addMinutes(base, 0);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 2000, 6, 5, 4, 3, 2, 1);
result = DateUtils.addMinutes(base, 1);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 2000, 6, 5, 4, 4, 2, 1);
result = DateUtils.addMinutes(base, -1);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 2000, 6, 5, 4, 2, 2, 1);
}
//-----------------------------------------------------------------------
public void testAddSeconds() throws Exception {
Date base = new Date(MILLIS_TEST);
Date result = DateUtils.addSeconds(base, 0);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 2000, 6, 5, 4, 3, 2, 1);
result = DateUtils.addSeconds(base, 1);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 2000, 6, 5, 4, 3, 3, 1);
result = DateUtils.addSeconds(base, -1);
assertNotSame(base, result);
assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
assertDate(result, 2000, 6, 5, 4, 3, 1, 1);
}
//-----------------------------------------------------------------------
public void testAddMilliseconds() {}
// Defects4J: flaky method
// public void testAddMilliseconds() throws Exception {
// Date base = new Date(MILLIS_TEST);
// Date result = DateUtils.addMilliseconds(base, 0);
// assertNotSame(base, result);
// assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
// assertDate(result, 2000, 6, 5, 4, 3, 2, 1);
//
// result = DateUtils.addMilliseconds(base, 1);
// assertNotSame(base, result);
// assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
// assertDate(result, 2000, 6, 5, 4, 3, 2, 2);
//
// result = DateUtils.addMilliseconds(base, -1);
// assertNotSame(base, result);
// assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
// assertDate(result, 2000, 6, 5, 4, 3, 2, 0);
// }
//-----------------------------------------------------------------------
public void testAddByField() {}
// Defects4J: flaky method
// public void testAddByField() throws Exception {
// Date base = new Date(MILLIS_TEST);
// Date result = DateUtils.add(base, Calendar.YEAR, 0);
// assertNotSame(base, result);
// assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
// assertDate(result, 2000, 6, 5, 4, 3, 2, 1);
//
// result = DateUtils.add(base, Calendar.YEAR, 1);
// assertNotSame(base, result);
// assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
// assertDate(result, 2001, 6, 5, 4, 3, 2, 1);
//
// result = DateUtils.add(base, Calendar.YEAR, -1);
// assertNotSame(base, result);
// assertDate(base, 2000, 6, 5, 4, 3, 2, 1);
// assertDate(result, 1999, 6, 5, 4, 3, 2, 1);
// }
//-----------------------------------------------------------------------
private void assertDate(Date date, int year, int month, int day, int hour, int min, int sec, int mil) throws Exception {
GregorianCalendar cal = new GregorianCalendar();
cal.setTime(date);
assertEquals(year, cal.get(Calendar.YEAR));
assertEquals(month, cal.get(Calendar.MONTH));
assertEquals(day, cal.get(Calendar.DAY_OF_MONTH));
assertEquals(hour, cal.get(Calendar.HOUR_OF_DAY));
assertEquals(min, cal.get(Calendar.MINUTE));
assertEquals(sec, cal.get(Calendar.SECOND));
assertEquals(mil, cal.get(Calendar.MILLISECOND));
}
//-----------------------------------------------------------------------
/**
* Tests various values with the round method
*/
public void testRound() throws Exception {
// tests for public static Date round(Date date, int field)
assertEquals("round year-1 failed",
dateParser.parse("January 1, 2002"),
DateUtils.round(date1, Calendar.YEAR));
assertEquals("round year-2 failed",
dateParser.parse("January 1, 2002"),
DateUtils.round(date2, Calendar.YEAR));
assertEquals("round month-1 failed",
dateParser.parse("February 1, 2002"),
DateUtils.round(date1, Calendar.MONTH));
assertEquals("round month-2 failed",
dateParser.parse("December 1, 2001"),
DateUtils.round(date2, Calendar.MONTH));
assertEquals("round semimonth-0 failed",
dateParser.parse("February 1, 2002"),
DateUtils.round(date0, DateUtils.SEMI_MONTH));
assertEquals("round semimonth-1 failed",
dateParser.parse("February 16, 2002"),
DateUtils.round(date1, DateUtils.SEMI_MONTH));
assertEquals("round semimonth-2 failed",
dateParser.parse("November 16, 2001"),
DateUtils.round(date2, DateUtils.SEMI_MONTH));
assertEquals("round date-1 failed",
dateParser.parse("February 13, 2002"),
DateUtils.round(date1, Calendar.DATE));
assertEquals("round date-2 failed",
dateParser.parse("November 18, 2001"),
DateUtils.round(date2, Calendar.DATE));
assertEquals("round hour-1 failed",
dateTimeParser.parse("February 12, 2002 13:00:00.000"),
DateUtils.round(date1, Calendar.HOUR));
assertEquals("round hour-2 failed",
dateTimeParser.parse("November 18, 2001 1:00:00.000"),
DateUtils.round(date2, Calendar.HOUR));
assertEquals("round minute-1 failed",
dateTimeParser.parse("February 12, 2002 12:35:00.000"),
DateUtils.round(date1, Calendar.MINUTE));
assertEquals("round minute-2 failed",
dateTimeParser.parse("November 18, 2001 1:23:00.000"),
DateUtils.round(date2, Calendar.MINUTE));
assertEquals("round second-1 failed",
dateTimeParser.parse("February 12, 2002 12:34:57.000"),
DateUtils.round(date1, Calendar.SECOND));
assertEquals("round second-2 failed",
dateTimeParser.parse("November 18, 2001 1:23:11.000"),
DateUtils.round(date2, Calendar.SECOND));
assertEquals("truncate ampm-1 failed",
dateTimeParser.parse("February 3, 2002 00:00:00.000"),
DateUtils.round(dateAmPm1, Calendar.AM_PM));
assertEquals("truncate ampm-2 failed",
dateTimeParser.parse("February 4, 2002 00:00:00.000"),
DateUtils.round(dateAmPm2, Calendar.AM_PM));
assertEquals("truncate ampm-3 failed",
dateTimeParser.parse("February 3, 2002 12:00:00.000"),
DateUtils.round(dateAmPm3, Calendar.AM_PM));
assertEquals("truncate ampm-4 failed",
dateTimeParser.parse("February 4, 2002 12:00:00.000"),
DateUtils.round(dateAmPm4, Calendar.AM_PM));
// tests for public static Date round(Object date, int field)
assertEquals("round year-1 failed",
dateParser.parse("January 1, 2002"),
DateUtils.round((Object) date1, Calendar.YEAR));
assertEquals("round year-2 failed",
dateParser.parse("January 1, 2002"),
DateUtils.round((Object) date2, Calendar.YEAR));
assertEquals("round month-1 failed",
dateParser.parse("February 1, 2002"),
DateUtils.round((Object) date1, Calendar.MONTH));
assertEquals("round month-2 failed",
dateParser.parse("December 1, 2001"),
DateUtils.round((Object) date2, Calendar.MONTH));
assertEquals("round semimonth-1 failed",
dateParser.parse("February 16, 2002"),
DateUtils.round((Object) date1, DateUtils.SEMI_MONTH));
assertEquals("round semimonth-2 failed",
dateParser.parse("November 16, 2001"),
DateUtils.round((Object) date2, DateUtils.SEMI_MONTH));
assertEquals("round date-1 failed",
dateParser.parse("February 13, 2002"),
DateUtils.round((Object) date1, Calendar.DATE));
assertEquals("round date-2 failed",
dateParser.parse("November 18, 2001"),
DateUtils.round((Object) date2, Calendar.DATE));
assertEquals("round hour-1 failed",
dateTimeParser.parse("February 12, 2002 13:00:00.000"),
DateUtils.round((Object) date1, Calendar.HOUR));
assertEquals("round hour-2 failed",
dateTimeParser.parse("November 18, 2001 1:00:00.000"),
DateUtils.round((Object) date2, Calendar.HOUR));
assertEquals("round minute-1 failed",
dateTimeParser.parse("February 12, 2002 12:35:00.000"),
DateUtils.round((Object) date1, Calendar.MINUTE));
assertEquals("round minute-2 failed",
dateTimeParser.parse("November 18, 2001 1:23:00.000"),
DateUtils.round((Object) date2, Calendar.MINUTE));
assertEquals("round second-1 failed",
dateTimeParser.parse("February 12, 2002 12:34:57.000"),
DateUtils.round((Object) date1, Calendar.SECOND));
assertEquals("round second-2 failed",
dateTimeParser.parse("November 18, 2001 1:23:11.000"),
DateUtils.round((Object) date2, Calendar.SECOND));
assertEquals("round calendar second-1 failed",
dateTimeParser.parse("February 12, 2002 12:34:57.000"),
DateUtils.round((Object) cal1, Calendar.SECOND));
assertEquals("round calendar second-2 failed",
dateTimeParser.parse("November 18, 2001 1:23:11.000"),
DateUtils.round((Object) cal2, Calendar.SECOND));
assertEquals("truncate ampm-1 failed",
dateTimeParser.parse("February 3, 2002 00:00:00.000"),
DateUtils.round((Object) dateAmPm1, Calendar.AM_PM));
assertEquals("truncate ampm-2 failed",
dateTimeParser.parse("February 4, 2002 00:00:00.000"),
DateUtils.round((Object) dateAmPm2, Calendar.AM_PM));
assertEquals("truncate ampm-3 failed",
dateTimeParser.parse("February 3, 2002 12:00:00.000"),
DateUtils.round((Object) dateAmPm3, Calendar.AM_PM));
assertEquals("truncate ampm-4 failed",
dateTimeParser.parse("February 4, 2002 12:00:00.000"),
DateUtils.round((Object) dateAmPm4, Calendar.AM_PM));
try {
DateUtils.round((Date) null, Calendar.SECOND);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateUtils.round((Calendar) null, Calendar.SECOND);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateUtils.round((Object) null, Calendar.SECOND);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateUtils.round("", Calendar.SECOND);
fail();
} catch (ClassCastException ex) {}
try {
DateUtils.round(date1, -9999);
fail();
} catch(IllegalArgumentException ex) {}
assertEquals("truncate ampm-1 failed",
dateTimeParser.parse("February 3, 2002 00:00:00.000"),
DateUtils.round((Object) calAmPm1, Calendar.AM_PM));
assertEquals("truncate ampm-2 failed",
dateTimeParser.parse("February 4, 2002 00:00:00.000"),
DateUtils.round((Object) calAmPm2, Calendar.AM_PM));
assertEquals("truncate ampm-3 failed",
dateTimeParser.parse("February 3, 2002 12:00:00.000"),
DateUtils.round((Object) calAmPm3, Calendar.AM_PM));
assertEquals("truncate ampm-4 failed",
dateTimeParser.parse("February 4, 2002 12:00:00.000"),
DateUtils.round((Object) calAmPm4, Calendar.AM_PM));
// Fix for http://issues.apache.org/bugzilla/show_bug.cgi?id=25560
// Test rounding across the beginning of daylight saving time
TimeZone.setDefault(zone);
dateTimeParser.setTimeZone(zone);
assertEquals("round MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 00:00:00.000"),
DateUtils.round(date4, Calendar.DATE));
assertEquals("round MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 00:00:00.000"),
DateUtils.round((Object) cal4, Calendar.DATE));
assertEquals("round MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 00:00:00.000"),
DateUtils.round(date5, Calendar.DATE));
assertEquals("round MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 00:00:00.000"),
DateUtils.round((Object) cal5, Calendar.DATE));
assertEquals("round MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 00:00:00.000"),
DateUtils.round(date6, Calendar.DATE));
assertEquals("round MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 00:00:00.000"),
DateUtils.round((Object) cal6, Calendar.DATE));
assertEquals("round MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 00:00:00.000"),
DateUtils.round(date7, Calendar.DATE));
assertEquals("round MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 00:00:00.000"),
DateUtils.round((Object) cal7, Calendar.DATE));
assertEquals("round MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 01:00:00.000"),
DateUtils.round(date4, Calendar.HOUR_OF_DAY));
assertEquals("round MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 01:00:00.000"),
DateUtils.round((Object) cal4, Calendar.HOUR_OF_DAY));
if (SystemUtils.isJavaVersionAtLeast(1.4f)) {
assertEquals("round MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 03:00:00.000"),
DateUtils.round(date5, Calendar.HOUR_OF_DAY));
assertEquals("round MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 03:00:00.000"),
DateUtils.round((Object) cal5, Calendar.HOUR_OF_DAY));
assertEquals("round MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 03:00:00.000"),
DateUtils.round(date6, Calendar.HOUR_OF_DAY));
assertEquals("round MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 03:00:00.000"),
DateUtils.round((Object) cal6, Calendar.HOUR_OF_DAY));
assertEquals("round MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 04:00:00.000"),
DateUtils.round(date7, Calendar.HOUR_OF_DAY));
assertEquals("round MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 04:00:00.000"),
DateUtils.round((Object) cal7, Calendar.HOUR_OF_DAY));
} else {
this.warn("WARNING: Some date rounding tests not run since the current version is " + SystemUtils.JAVA_VERSION);
}
TimeZone.setDefault(defaultZone);
dateTimeParser.setTimeZone(defaultZone);
}
/**
* Tests the Changes Made by LANG-346 to the DateUtils.modify() private method invoked
* by DateUtils.round().
*/
public void testRoundLang346() throws Exception
{
TimeZone.setDefault(defaultZone);
dateTimeParser.setTimeZone(defaultZone);
Calendar testCalendar = Calendar.getInstance();
testCalendar.set(2007, 6, 2, 8, 8, 50);
Date date = testCalendar.getTime();
assertEquals("Minute Round Up Failed",
dateTimeParser.parse("July 2, 2007 08:09:00.000"),
DateUtils.round(date, Calendar.MINUTE));
testCalendar.set(2007, 6, 2, 8, 8, 20);
date = testCalendar.getTime();
assertEquals("Minute No Round Failed",
dateTimeParser.parse("July 2, 2007 08:08:00.000"),
DateUtils.round(date, Calendar.MINUTE));
testCalendar.set(2007, 6, 2, 8, 8, 50);
testCalendar.set(Calendar.MILLISECOND, 600);
date = testCalendar.getTime();
assertEquals("Second Round Up with 600 Milli Seconds Failed",
dateTimeParser.parse("July 2, 2007 08:08:51.000"),
DateUtils.round(date, Calendar.SECOND));
testCalendar.set(2007, 6, 2, 8, 8, 50);
testCalendar.set(Calendar.MILLISECOND, 200);
date = testCalendar.getTime();
assertEquals("Second Round Down with 200 Milli Seconds Failed",
dateTimeParser.parse("July 2, 2007 08:08:50.000"),
DateUtils.round(date, Calendar.SECOND));
testCalendar.set(2007, 6, 2, 8, 8, 20);
testCalendar.set(Calendar.MILLISECOND, 600);
date = testCalendar.getTime();
assertEquals("Second Round Up with 200 Milli Seconds Failed",
dateTimeParser.parse("July 2, 2007 08:08:21.000"),
DateUtils.round(date, Calendar.SECOND));
testCalendar.set(2007, 6, 2, 8, 8, 20);
testCalendar.set(Calendar.MILLISECOND, 200);
date = testCalendar.getTime();
assertEquals("Second Round Down with 200 Milli Seconds Failed",
dateTimeParser.parse("July 2, 2007 08:08:20.000"),
DateUtils.round(date, Calendar.SECOND));
testCalendar.set(2007, 6, 2, 8, 8, 50);
date = testCalendar.getTime();
assertEquals("Hour Round Down Failed",
dateTimeParser.parse("July 2, 2007 08:00:00.000"),
DateUtils.round(date, Calendar.HOUR));
testCalendar.set(2007, 6, 2, 8, 31, 50);
date = testCalendar.getTime();
assertEquals("Hour Round Up Failed",
dateTimeParser.parse("July 2, 2007 09:00:00.000"),
DateUtils.round(date, Calendar.HOUR));
}
/**
* Tests various values with the trunc method
*/
public void testTruncate() throws Exception {
// tests public static Date truncate(Date date, int field)
assertEquals("truncate year-1 failed",
dateParser.parse("January 1, 2002"),
DateUtils.truncate(date1, Calendar.YEAR));
assertEquals("truncate year-2 failed",
dateParser.parse("January 1, 2001"),
DateUtils.truncate(date2, Calendar.YEAR));
assertEquals("truncate month-1 failed",
dateParser.parse("February 1, 2002"),
DateUtils.truncate(date1, Calendar.MONTH));
assertEquals("truncate month-2 failed",
dateParser.parse("November 1, 2001"),
DateUtils.truncate(date2, Calendar.MONTH));
assertEquals("truncate semimonth-1 failed",
dateParser.parse("February 1, 2002"),
DateUtils.truncate(date1, DateUtils.SEMI_MONTH));
assertEquals("truncate semimonth-2 failed",
dateParser.parse("November 16, 2001"),
DateUtils.truncate(date2, DateUtils.SEMI_MONTH));
assertEquals("truncate date-1 failed",
dateParser.parse("February 12, 2002"),
DateUtils.truncate(date1, Calendar.DATE));
assertEquals("truncate date-2 failed",
dateParser.parse("November 18, 2001"),
DateUtils.truncate(date2, Calendar.DATE));
assertEquals("truncate hour-1 failed",
dateTimeParser.parse("February 12, 2002 12:00:00.000"),
DateUtils.truncate(date1, Calendar.HOUR));
assertEquals("truncate hour-2 failed",
dateTimeParser.parse("November 18, 2001 1:00:00.000"),
DateUtils.truncate(date2, Calendar.HOUR));
assertEquals("truncate minute-1 failed",
dateTimeParser.parse("February 12, 2002 12:34:00.000"),
DateUtils.truncate(date1, Calendar.MINUTE));
assertEquals("truncate minute-2 failed",
dateTimeParser.parse("November 18, 2001 1:23:00.000"),
DateUtils.truncate(date2, Calendar.MINUTE));
assertEquals("truncate second-1 failed",
dateTimeParser.parse("February 12, 2002 12:34:56.000"),
DateUtils.truncate(date1, Calendar.SECOND));
assertEquals("truncate second-2 failed",
dateTimeParser.parse("November 18, 2001 1:23:11.000"),
DateUtils.truncate(date2, Calendar.SECOND));
assertEquals("truncate ampm-1 failed",
dateTimeParser.parse("February 3, 2002 00:00:00.000"),
DateUtils.truncate(dateAmPm1, Calendar.AM_PM));
assertEquals("truncate ampm-2 failed",
dateTimeParser.parse("February 3, 2002 00:00:00.000"),
DateUtils.truncate(dateAmPm2, Calendar.AM_PM));
assertEquals("truncate ampm-3 failed",
dateTimeParser.parse("February 3, 2002 12:00:00.000"),
DateUtils.truncate(dateAmPm3, Calendar.AM_PM));
assertEquals("truncate ampm-4 failed",
dateTimeParser.parse("February 3, 2002 12:00:00.000"),
DateUtils.truncate(dateAmPm4, Calendar.AM_PM));
// tests public static Date truncate(Object date, int field)
assertEquals("truncate year-1 failed",
dateParser.parse("January 1, 2002"),
DateUtils.truncate((Object) date1, Calendar.YEAR));
assertEquals("truncate year-2 failed",
dateParser.parse("January 1, 2001"),
DateUtils.truncate((Object) date2, Calendar.YEAR));
assertEquals("truncate month-1 failed",
dateParser.parse("February 1, 2002"),
DateUtils.truncate((Object) date1, Calendar.MONTH));
assertEquals("truncate month-2 failed",
dateParser.parse("November 1, 2001"),
DateUtils.truncate((Object) date2, Calendar.MONTH));
assertEquals("truncate semimonth-1 failed",
dateParser.parse("February 1, 2002"),
DateUtils.truncate((Object) date1, DateUtils.SEMI_MONTH));
assertEquals("truncate semimonth-2 failed",
dateParser.parse("November 16, 2001"),
DateUtils.truncate((Object) date2, DateUtils.SEMI_MONTH));
assertEquals("truncate date-1 failed",
dateParser.parse("February 12, 2002"),
DateUtils.truncate((Object) date1, Calendar.DATE));
assertEquals("truncate date-2 failed",
dateParser.parse("November 18, 2001"),
DateUtils.truncate((Object) date2, Calendar.DATE));
assertEquals("truncate hour-1 failed",
dateTimeParser.parse("February 12, 2002 12:00:00.000"),
DateUtils.truncate((Object) date1, Calendar.HOUR));
assertEquals("truncate hour-2 failed",
dateTimeParser.parse("November 18, 2001 1:00:00.000"),
DateUtils.truncate((Object) date2, Calendar.HOUR));
assertEquals("truncate minute-1 failed",
dateTimeParser.parse("February 12, 2002 12:34:00.000"),
DateUtils.truncate((Object) date1, Calendar.MINUTE));
assertEquals("truncate minute-2 failed",
dateTimeParser.parse("November 18, 2001 1:23:00.000"),
DateUtils.truncate((Object) date2, Calendar.MINUTE));
assertEquals("truncate second-1 failed",
dateTimeParser.parse("February 12, 2002 12:34:56.000"),
DateUtils.truncate((Object) date1, Calendar.SECOND));
assertEquals("truncate second-2 failed",
dateTimeParser.parse("November 18, 2001 1:23:11.000"),
DateUtils.truncate((Object) date2, Calendar.SECOND));
assertEquals("truncate ampm-1 failed",
dateTimeParser.parse("February 3, 2002 00:00:00.000"),
DateUtils.truncate((Object) dateAmPm1, Calendar.AM_PM));
assertEquals("truncate ampm-2 failed",
dateTimeParser.parse("February 3, 2002 00:00:00.000"),
DateUtils.truncate((Object) dateAmPm2, Calendar.AM_PM));
assertEquals("truncate ampm-3 failed",
dateTimeParser.parse("February 3, 2002 12:00:00.000"),
DateUtils.truncate((Object) dateAmPm3, Calendar.AM_PM));
assertEquals("truncate ampm-4 failed",
dateTimeParser.parse("February 3, 2002 12:00:00.000"),
DateUtils.truncate((Object) dateAmPm4, Calendar.AM_PM));
assertEquals("truncate calendar second-1 failed",
dateTimeParser.parse("February 12, 2002 12:34:56.000"),
DateUtils.truncate((Object) cal1, Calendar.SECOND));
assertEquals("truncate calendar second-2 failed",
dateTimeParser.parse("November 18, 2001 1:23:11.000"),
DateUtils.truncate((Object) cal2, Calendar.SECOND));
assertEquals("truncate ampm-1 failed",
dateTimeParser.parse("February 3, 2002 00:00:00.000"),
DateUtils.truncate((Object) calAmPm1, Calendar.AM_PM));
assertEquals("truncate ampm-2 failed",
dateTimeParser.parse("February 3, 2002 00:00:00.000"),
DateUtils.truncate((Object) calAmPm2, Calendar.AM_PM));
assertEquals("truncate ampm-3 failed",
dateTimeParser.parse("February 3, 2002 12:00:00.000"),
DateUtils.truncate((Object) calAmPm3, Calendar.AM_PM));
assertEquals("truncate ampm-4 failed",
dateTimeParser.parse("February 3, 2002 12:00:00.000"),
DateUtils.truncate((Object) calAmPm4, Calendar.AM_PM));
try {
DateUtils.truncate((Date) null, Calendar.SECOND);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateUtils.truncate((Calendar) null, Calendar.SECOND);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateUtils.truncate((Object) null, Calendar.SECOND);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateUtils.truncate("", Calendar.SECOND);
fail();
} catch (ClassCastException ex) {}
// Fix for http://issues.apache.org/bugzilla/show_bug.cgi?id=25560
// Test truncate across beginning of daylight saving time
TimeZone.setDefault(zone);
dateTimeParser.setTimeZone(zone);
assertEquals("truncate MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 00:00:00.000"),
DateUtils.truncate(date3, Calendar.DATE));
assertEquals("truncate MET date across DST change-over",
dateTimeParser.parse("March 30, 2003 00:00:00.000"),
DateUtils.truncate((Object) cal3, Calendar.DATE));
// Test truncate across end of daylight saving time
assertEquals("truncate MET date across DST change-over",
dateTimeParser.parse("October 26, 2003 00:00:00.000"),
DateUtils.truncate(date8, Calendar.DATE));
assertEquals("truncate MET date across DST change-over",
dateTimeParser.parse("October 26, 2003 00:00:00.000"),
DateUtils.truncate((Object) cal8, Calendar.DATE));
TimeZone.setDefault(defaultZone);
dateTimeParser.setTimeZone(defaultZone);
// Bug 31395, large dates
Date endOfTime = new Date(Long.MAX_VALUE); // fyi: Sun Aug 17 07:12:55 CET 292278994 -- 807 millis
GregorianCalendar endCal = new GregorianCalendar();
endCal.setTime(endOfTime);
try {
DateUtils.truncate(endCal, Calendar.DATE);
fail();
} catch (ArithmeticException ex) {}
endCal.set(Calendar.YEAR, 280000001);
try {
DateUtils.truncate(endCal, Calendar.DATE);
fail();
} catch (ArithmeticException ex) {}
endCal.set(Calendar.YEAR, 280000000);
Calendar cal = DateUtils.truncate(endCal, Calendar.DATE);
assertEquals(0, cal.get(Calendar.HOUR));
}
/**
* Tests for LANG-59
*
* see http://issues.apache.org/jira/browse/LANG-59
*/
public void testTruncateLang59() throws Exception {
if (!SystemUtils.isJavaVersionAtLeast(1.4f)) {
this.warn("WARNING: Test for LANG-59 not run since the current version is " + SystemUtils.JAVA_VERSION);
return;
}
// Set TimeZone to Mountain Time
TimeZone MST_MDT = TimeZone.getTimeZone("MST7MDT");
TimeZone.setDefault(MST_MDT);
DateFormat format = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss.SSS z");
format.setTimeZone(MST_MDT);
Date oct31_01MDT = new Date(1099206000000L);
Date oct31MDT = new Date(oct31_01MDT.getTime() - 3600000L); // - 1 hour
Date oct31_01_02MDT = new Date(oct31_01MDT.getTime() + 120000L); // + 2 minutes
Date oct31_01_02_03MDT = new Date(oct31_01_02MDT.getTime() + 3000L); // + 3 seconds
Date oct31_01_02_03_04MDT = new Date(oct31_01_02_03MDT.getTime() + 4L); // + 4 milliseconds
assertEquals("Check 00:00:00.000", "2004-10-31 00:00:00.000 MDT", format.format(oct31MDT));
assertEquals("Check 01:00:00.000", "2004-10-31 01:00:00.000 MDT", format.format(oct31_01MDT));
assertEquals("Check 01:02:00.000", "2004-10-31 01:02:00.000 MDT", format.format(oct31_01_02MDT));
assertEquals("Check 01:02:03.000", "2004-10-31 01:02:03.000 MDT", format.format(oct31_01_02_03MDT));
assertEquals("Check 01:02:03.004", "2004-10-31 01:02:03.004 MDT", format.format(oct31_01_02_03_04MDT));
// ------- Demonstrate Problem -------
Calendar gval = Calendar.getInstance();
gval.setTime(new Date(oct31_01MDT.getTime()));
gval.set(Calendar.MINUTE, gval.get(Calendar.MINUTE)); // set minutes to the same value
assertEquals("Demonstrate Problem", gval.getTime().getTime(), oct31_01MDT.getTime() + 3600000L);
// ---------- Test Truncate ----------
assertEquals("Truncate Calendar.MILLISECOND",
oct31_01_02_03_04MDT, DateUtils.truncate(oct31_01_02_03_04MDT, Calendar.MILLISECOND));
assertEquals("Truncate Calendar.SECOND",
oct31_01_02_03MDT, DateUtils.truncate(oct31_01_02_03_04MDT, Calendar.SECOND));
assertEquals("Truncate Calendar.MINUTE",
oct31_01_02MDT, DateUtils.truncate(oct31_01_02_03_04MDT, Calendar.MINUTE));
assertEquals("Truncate Calendar.HOUR_OF_DAY",
oct31_01MDT, DateUtils.truncate(oct31_01_02_03_04MDT, Calendar.HOUR_OF_DAY));
assertEquals("Truncate Calendar.HOUR",
oct31_01MDT, DateUtils.truncate(oct31_01_02_03_04MDT, Calendar.HOUR));
assertEquals("Truncate Calendar.DATE",
oct31MDT, DateUtils.truncate(oct31_01_02_03_04MDT, Calendar.DATE));
// ---------- Test Round (down) ----------
assertEquals("Round Calendar.MILLISECOND",
oct31_01_02_03_04MDT, DateUtils.round(oct31_01_02_03_04MDT, Calendar.MILLISECOND));
assertEquals("Round Calendar.SECOND",
oct31_01_02_03MDT, DateUtils.round(oct31_01_02_03_04MDT, Calendar.SECOND));
assertEquals("Round Calendar.MINUTE",
oct31_01_02MDT, DateUtils.round(oct31_01_02_03_04MDT, Calendar.MINUTE));
assertEquals("Round Calendar.HOUR_OF_DAY",
oct31_01MDT, DateUtils.round(oct31_01_02_03_04MDT, Calendar.HOUR_OF_DAY));
assertEquals("Round Calendar.HOUR",
oct31_01MDT, DateUtils.round(oct31_01_02_03_04MDT, Calendar.HOUR));
assertEquals("Round Calendar.DATE",
oct31MDT, DateUtils.round(oct31_01_02_03_04MDT, Calendar.DATE));
// restore default time zone
TimeZone.setDefault(defaultZone);
}
/**
* Tests the iterator exceptions
*/
public void testIteratorEx() throws Exception {
try {
DateUtils.iterator(Calendar.getInstance(), -9999);
} catch (IllegalArgumentException ex) {}
try {
DateUtils.iterator((Date) null, DateUtils.RANGE_WEEK_CENTER);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateUtils.iterator((Calendar) null, DateUtils.RANGE_WEEK_CENTER);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateUtils.iterator((Object) null, DateUtils.RANGE_WEEK_CENTER);
fail();
} catch (IllegalArgumentException ex) {}
try {
DateUtils.iterator("", DateUtils.RANGE_WEEK_CENTER);
fail();
} catch (ClassCastException ex) {}
}
/**
* Tests the calendar iterator for week ranges
*/
public void testWeekIterator() throws Exception {
Calendar now = Calendar.getInstance();
for (int i = 0; i< 7; i++) {
Calendar today = DateUtils.truncate(now, Calendar.DATE);
Calendar sunday = DateUtils.truncate(now, Calendar.DATE);
sunday.add(Calendar.DATE, 1 - sunday.get(Calendar.DAY_OF_WEEK));
Calendar monday = DateUtils.truncate(now, Calendar.DATE);
if (monday.get(Calendar.DAY_OF_WEEK) == 1) {
//This is sunday... roll back 6 days
monday.add(Calendar.DATE, -6);
} else {
monday.add(Calendar.DATE, 2 - monday.get(Calendar.DAY_OF_WEEK));
}
Calendar centered = DateUtils.truncate(now, Calendar.DATE);
centered.add(Calendar.DATE, -3);
Iterator it = DateUtils.iterator(now, DateUtils.RANGE_WEEK_SUNDAY);
assertWeekIterator(it, sunday);
it = DateUtils.iterator(now, DateUtils.RANGE_WEEK_MONDAY);
assertWeekIterator(it, monday);
it = DateUtils.iterator(now, DateUtils.RANGE_WEEK_RELATIVE);
assertWeekIterator(it, today);
it = DateUtils.iterator(now, DateUtils.RANGE_WEEK_CENTER);
assertWeekIterator(it, centered);
it = DateUtils.iterator((Object) now, DateUtils.RANGE_WEEK_CENTER);
assertWeekIterator(it, centered);
it = DateUtils.iterator((Object) now.getTime(), DateUtils.RANGE_WEEK_CENTER);
assertWeekIterator(it, centered);
try {
it.next();
fail();
} catch (NoSuchElementException ex) {}
it = DateUtils.iterator(now, DateUtils.RANGE_WEEK_CENTER);
it.next();
try {
it.remove();
} catch( UnsupportedOperationException ex) {}
now.add(Calendar.DATE,1);
}
}
/**
* Tests the calendar iterator for month-based ranges
*/
public void testMonthIterator() throws Exception {
Iterator it = DateUtils.iterator(date1, DateUtils.RANGE_MONTH_SUNDAY);
assertWeekIterator(it,
dateParser.parse("January 27, 2002"),
dateParser.parse("March 2, 2002"));
it = DateUtils.iterator(date1, DateUtils.RANGE_MONTH_MONDAY);
assertWeekIterator(it,
dateParser.parse("January 28, 2002"),
dateParser.parse("March 3, 2002"));
it = DateUtils.iterator(date2, DateUtils.RANGE_MONTH_SUNDAY);
assertWeekIterator(it,
dateParser.parse("October 28, 2001"),
dateParser.parse("December 1, 2001"));
it = DateUtils.iterator(date2, DateUtils.RANGE_MONTH_MONDAY);
assertWeekIterator(it,
dateParser.parse("October 29, 2001"),
dateParser.parse("December 2, 2001"));
}
/**
* This checks that this is a 7 element iterator of Calendar objects
* that are dates (no time), and exactly 1 day spaced after each other.
*/
private static void assertWeekIterator(Iterator it, Calendar start) {
Calendar end = (Calendar) start.clone();
end.add(Calendar.DATE, 6);
assertWeekIterator(it, start, end);
}
/**
* Convenience method for when working with Date objects
*/
private static void assertWeekIterator(Iterator it, Date start, Date end) {
Calendar calStart = Calendar.getInstance();
calStart.setTime(start);
Calendar calEnd = Calendar.getInstance();
calEnd.setTime(end);
assertWeekIterator(it, calStart, calEnd);
}
/**
* This checks that this is a 7 divisble iterator of Calendar objects
* that are dates (no time), and exactly 1 day spaced after each other
* (in addition to the proper start and stop dates)
*/
private static void assertWeekIterator(Iterator it, Calendar start, Calendar end) {
Calendar cal = (Calendar) it.next();
assertEquals("", start, cal, 0);
Calendar last = null;
int count = 1;
while (it.hasNext()) {
//Check this is just a date (no time component)
assertEquals("", cal, DateUtils.truncate(cal, Calendar.DATE), 0);
last = cal;
cal = (Calendar) it.next();
count++;
//Check that this is one day more than the last date
last.add(Calendar.DATE, 1);
assertEquals("", last, cal, 0);
}
if (count % 7 != 0) {
throw new AssertionFailedError("There were " + count + " days in this iterator");
}
assertEquals("", end, cal, 0);
}
/**
* Used to check that Calendar objects are close enough
* delta is in milliseconds
*/
private static void assertEquals(String message, Calendar cal1, Calendar cal2, long delta) {
if (Math.abs(cal1.getTime().getTime() - cal2.getTime().getTime()) > delta) {
throw new AssertionFailedError(
message + " expected " + cal1.getTime() + " but got " + cal2.getTime());
}
}
void warn(String msg) {
System.err.println(msg);
}
}
|
|
@Test(expected=ConvergenceException.class)
public void testIssue631() {
final UnivariateRealFunction f = new UnivariateRealFunction() {
/** {@inheritDoc} */
public double value(double x) {
return Math.exp(x) - Math.pow(Math.PI, 3.0);
}
};
final UnivariateRealSolver solver = new RegulaFalsiSolver();
final double root = solver.solve(3624, f, 1, 10);
Assert.assertEquals(3.4341896575482003, root, 1e-15);
} | org.apache.commons.math.analysis.solvers.RegulaFalsiSolverTest::testIssue631 | src/test/java/org/apache/commons/math/analysis/solvers/RegulaFalsiSolverTest.java | 55 | src/test/java/org/apache/commons/math/analysis/solvers/RegulaFalsiSolverTest.java | testIssue631 | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.math.analysis.solvers;
import org.apache.commons.math.analysis.UnivariateRealFunction;
import org.apache.commons.math.exception.ConvergenceException;
import org.junit.Test;
import org.junit.Assert;
/**
* Test case for {@link RegulaFalsiSolver Regula Falsi} solver.
*
* @version $Id$
*/
public final class RegulaFalsiSolverTest extends BaseSecantSolverAbstractTest {
/** {@inheritDoc} */
protected UnivariateRealSolver getSolver() {
return new RegulaFalsiSolver();
}
/** {@inheritDoc} */
protected int[] getQuinticEvalCounts() {
// While the Regula Falsi method guarantees convergence, convergence
// may be extremely slow. The last test case does not converge within
// even a million iterations. As such, it was disabled.
return new int[] {3, 7, 8, 19, 18, 11, 67, 55, 288, 151, -1};
}
@Test(expected=ConvergenceException.class)
public void testIssue631() {
final UnivariateRealFunction f = new UnivariateRealFunction() {
/** {@inheritDoc} */
public double value(double x) {
return Math.exp(x) - Math.pow(Math.PI, 3.0);
}
};
final UnivariateRealSolver solver = new RegulaFalsiSolver();
final double root = solver.solve(3624, f, 1, 10);
Assert.assertEquals(3.4341896575482003, root, 1e-15);
}
} | // You are a professional Java test case writer, please create a test case named `testIssue631` for the issue `Math-MATH-631`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Math-MATH-631
//
// ## Issue-Title:
// "RegulaFalsiSolver" failure
//
// ## Issue-Description:
//
// The following unit test:
//
//
//
//
// ```
// @Test
// public void testBug() {
// final UnivariateRealFunction f = new UnivariateRealFunction() {
// @Override
// public double value(double x) {
// return Math.exp(x) - Math.pow(Math.PI, 3.0);
// }
// };
//
// UnivariateRealSolver solver = new RegulaFalsiSolver();
// double root = solver.solve(100, f, 1, 10);
// }
//
// ```
//
//
// fails with
//
//
//
//
// ```
// illegal state: maximal count (100) exceeded: evaluations
//
// ```
//
//
// Using "PegasusSolver", the answer is found after 17 evaluations.
//
//
//
//
//
@Test(expected=ConvergenceException.class)
public void testIssue631() {
| 55 | 48 | 43 | src/test/java/org/apache/commons/math/analysis/solvers/RegulaFalsiSolverTest.java | src/test/java | ```markdown
## Issue-ID: Math-MATH-631
## Issue-Title:
"RegulaFalsiSolver" failure
## Issue-Description:
The following unit test:
```
@Test
public void testBug() {
final UnivariateRealFunction f = new UnivariateRealFunction() {
@Override
public double value(double x) {
return Math.exp(x) - Math.pow(Math.PI, 3.0);
}
};
UnivariateRealSolver solver = new RegulaFalsiSolver();
double root = solver.solve(100, f, 1, 10);
}
```
fails with
```
illegal state: maximal count (100) exceeded: evaluations
```
Using "PegasusSolver", the answer is found after 17 evaluations.
```
You are a professional Java test case writer, please create a test case named `testIssue631` for the issue `Math-MATH-631`, utilizing the provided issue report information and the following function signature.
```java
@Test(expected=ConvergenceException.class)
public void testIssue631() {
```
| 43 | [
"org.apache.commons.math.analysis.solvers.BaseSecantSolver"
] | 8f16d7e40d63ddcd947a4602c478620d318758113b67e8e11846cf96476d52e7 | @Test(expected=ConvergenceException.class)
public void testIssue631() | // You are a professional Java test case writer, please create a test case named `testIssue631` for the issue `Math-MATH-631`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Math-MATH-631
//
// ## Issue-Title:
// "RegulaFalsiSolver" failure
//
// ## Issue-Description:
//
// The following unit test:
//
//
//
//
// ```
// @Test
// public void testBug() {
// final UnivariateRealFunction f = new UnivariateRealFunction() {
// @Override
// public double value(double x) {
// return Math.exp(x) - Math.pow(Math.PI, 3.0);
// }
// };
//
// UnivariateRealSolver solver = new RegulaFalsiSolver();
// double root = solver.solve(100, f, 1, 10);
// }
//
// ```
//
//
// fails with
//
//
//
//
// ```
// illegal state: maximal count (100) exceeded: evaluations
//
// ```
//
//
// Using "PegasusSolver", the answer is found after 17 evaluations.
//
//
//
//
//
| Math | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.math.analysis.solvers;
import org.apache.commons.math.analysis.UnivariateRealFunction;
import org.apache.commons.math.exception.ConvergenceException;
import org.junit.Test;
import org.junit.Assert;
/**
* Test case for {@link RegulaFalsiSolver Regula Falsi} solver.
*
* @version $Id$
*/
public final class RegulaFalsiSolverTest extends BaseSecantSolverAbstractTest {
/** {@inheritDoc} */
protected UnivariateRealSolver getSolver() {
return new RegulaFalsiSolver();
}
/** {@inheritDoc} */
protected int[] getQuinticEvalCounts() {
// While the Regula Falsi method guarantees convergence, convergence
// may be extremely slow. The last test case does not converge within
// even a million iterations. As such, it was disabled.
return new int[] {3, 7, 8, 19, 18, 11, 67, 55, 288, 151, -1};
}
@Test(expected=ConvergenceException.class)
public void testIssue631() {
final UnivariateRealFunction f = new UnivariateRealFunction() {
/** {@inheritDoc} */
public double value(double x) {
return Math.exp(x) - Math.pow(Math.PI, 3.0);
}
};
final UnivariateRealSolver solver = new RegulaFalsiSolver();
final double root = solver.solve(3624, f, 1, 10);
Assert.assertEquals(3.4341896575482003, root, 1e-15);
}
} |
||
@Test
public void supportsBOMinFiles() throws IOException {
// test files from http://www.i18nl10n.com/korean/utftest/
File in = getFile("/bomtests/bom_utf16be.html");
Document doc = Jsoup.parse(in, null, "http://example.com");
assertTrue(doc.title().contains("UTF-16BE"));
assertTrue(doc.text().contains("가각갂갃간갅"));
in = getFile("/bomtests/bom_utf16le.html");
doc = Jsoup.parse(in, null, "http://example.com");
assertTrue(doc.title().contains("UTF-16LE"));
assertTrue(doc.text().contains("가각갂갃간갅"));
in = getFile("/bomtests/bom_utf32be.html");
doc = Jsoup.parse(in, null, "http://example.com");
assertTrue(doc.title().contains("UTF-32BE"));
assertTrue(doc.text().contains("가각갂갃간갅"));
in = getFile("/bomtests/bom_utf32le.html");
doc = Jsoup.parse(in, null, "http://example.com");
assertTrue(doc.title().contains("UTF-32LE"));
assertTrue(doc.text().contains("가각갂갃간갅"));
} | org.jsoup.helper.DataUtilTest::supportsBOMinFiles | src/test/java/org/jsoup/helper/DataUtilTest.java | 125 | src/test/java/org/jsoup/helper/DataUtilTest.java | supportsBOMinFiles | package org.jsoup.helper;
import java.io.File;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.parser.Parser;
import org.junit.Test;
import java.nio.ByteBuffer;
import java.nio.charset.Charset;
import static org.jsoup.integration.ParseTest.getFile;
import static org.junit.Assert.*;
public class DataUtilTest {
@Test
public void testCharset() {
assertEquals("utf-8", DataUtil.getCharsetFromContentType("text/html;charset=utf-8 "));
assertEquals("UTF-8", DataUtil.getCharsetFromContentType("text/html; charset=UTF-8"));
assertEquals("ISO-8859-1", DataUtil.getCharsetFromContentType("text/html; charset=ISO-8859-1"));
assertEquals(null, DataUtil.getCharsetFromContentType("text/html"));
assertEquals(null, DataUtil.getCharsetFromContentType(null));
assertEquals(null, DataUtil.getCharsetFromContentType("text/html;charset=Unknown"));
}
@Test public void testQuotedCharset() {
assertEquals("utf-8", DataUtil.getCharsetFromContentType("text/html; charset=\"utf-8\""));
assertEquals("UTF-8", DataUtil.getCharsetFromContentType("text/html;charset=\"UTF-8\""));
assertEquals("ISO-8859-1", DataUtil.getCharsetFromContentType("text/html; charset=\"ISO-8859-1\""));
assertEquals(null, DataUtil.getCharsetFromContentType("text/html; charset=\"Unsupported\""));
assertEquals("UTF-8", DataUtil.getCharsetFromContentType("text/html; charset='UTF-8'"));
}
@Test public void discardsSpuriousByteOrderMark() {
String html = "\uFEFF<html><head><title>One</title></head><body>Two</body></html>";
ByteBuffer buffer = Charset.forName("UTF-8").encode(html);
Document doc = DataUtil.parseByteData(buffer, "UTF-8", "http://foo.com/", Parser.htmlParser());
assertEquals("One", doc.head().text());
}
@Test public void discardsSpuriousByteOrderMarkWhenNoCharsetSet() {
String html = "\uFEFF<html><head><title>One</title></head><body>Two</body></html>";
ByteBuffer buffer = Charset.forName("UTF-8").encode(html);
Document doc = DataUtil.parseByteData(buffer, null, "http://foo.com/", Parser.htmlParser());
assertEquals("One", doc.head().text());
assertEquals("UTF-8", doc.outputSettings().charset().displayName());
}
@Test
public void shouldNotThrowExceptionOnEmptyCharset() {
assertEquals(null, DataUtil.getCharsetFromContentType("text/html; charset="));
assertEquals(null, DataUtil.getCharsetFromContentType("text/html; charset=;"));
}
@Test
public void shouldSelectFirstCharsetOnWeirdMultileCharsetsInMetaTags() {
assertEquals("ISO-8859-1", DataUtil.getCharsetFromContentType("text/html; charset=ISO-8859-1, charset=1251"));
}
@Test
public void shouldCorrectCharsetForDuplicateCharsetString() {
assertEquals("iso-8859-1", DataUtil.getCharsetFromContentType("text/html; charset=charset=iso-8859-1"));
}
@Test
public void shouldReturnNullForIllegalCharsetNames() {
assertEquals(null, DataUtil.getCharsetFromContentType("text/html; charset=$HJKDF§$/("));
}
@Test
public void generatesMimeBoundaries() {
String m1 = DataUtil.mimeBoundary();
String m2 = DataUtil.mimeBoundary();
assertEquals(DataUtil.boundaryLength, m1.length());
assertEquals(DataUtil.boundaryLength, m2.length());
assertNotSame(m1, m2);
}
@Test
public void wrongMetaCharsetFallback() {
try {
final byte[] input = "<html><head><meta charset=iso-8></head><body></body></html>".getBytes("UTF-8");
final ByteBuffer inBuffer = ByteBuffer.wrap(input);
Document doc = DataUtil.parseByteData(inBuffer, null, "http://example.com", Parser.htmlParser());
final String expected = "<html>\n" +
" <head>\n" +
" <meta charset=\"iso-8\">\n" +
" </head>\n" +
" <body></body>\n" +
"</html>";
assertEquals(expected, doc.toString());
} catch( UnsupportedEncodingException ex ) {
fail(ex.getMessage());
}
}
@Test
public void supportsBOMinFiles() throws IOException {
// test files from http://www.i18nl10n.com/korean/utftest/
File in = getFile("/bomtests/bom_utf16be.html");
Document doc = Jsoup.parse(in, null, "http://example.com");
assertTrue(doc.title().contains("UTF-16BE"));
assertTrue(doc.text().contains("가각갂갃간갅"));
in = getFile("/bomtests/bom_utf16le.html");
doc = Jsoup.parse(in, null, "http://example.com");
assertTrue(doc.title().contains("UTF-16LE"));
assertTrue(doc.text().contains("가각갂갃간갅"));
in = getFile("/bomtests/bom_utf32be.html");
doc = Jsoup.parse(in, null, "http://example.com");
assertTrue(doc.title().contains("UTF-32BE"));
assertTrue(doc.text().contains("가각갂갃간갅"));
in = getFile("/bomtests/bom_utf32le.html");
doc = Jsoup.parse(in, null, "http://example.com");
assertTrue(doc.title().contains("UTF-32LE"));
assertTrue(doc.text().contains("가각갂갃간갅"));
}
} | // You are a professional Java test case writer, please create a test case named `supportsBOMinFiles` for the issue `Jsoup-695`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Jsoup-695
//
// ## Issue-Title:
// UTF16 streams with BOM are processed as UTF-8
//
// ## Issue-Description:
// The handling of the character encoding in org.jsoup.helper.DataUtil.parseByteData(...) is bugged when the input is an UTF16 stream with unicode BOM. This method does a check for presence of a BOM and, if it finds one, incorrectly assumes that this was a UTF-8 BOM. To fix this, the code would have to check the raw BOM bytes as the distinction between the various BOMs is lost after conversion to characters. See also: <http://unicode.org/faq/utf_bom.html#bom4>
//
//
//
//
@Test
public void supportsBOMinFiles() throws IOException {
| 125 | 50 | 103 | src/test/java/org/jsoup/helper/DataUtilTest.java | src/test/java | ```markdown
## Issue-ID: Jsoup-695
## Issue-Title:
UTF16 streams with BOM are processed as UTF-8
## Issue-Description:
The handling of the character encoding in org.jsoup.helper.DataUtil.parseByteData(...) is bugged when the input is an UTF16 stream with unicode BOM. This method does a check for presence of a BOM and, if it finds one, incorrectly assumes that this was a UTF-8 BOM. To fix this, the code would have to check the raw BOM bytes as the distinction between the various BOMs is lost after conversion to characters. See also: <http://unicode.org/faq/utf_bom.html#bom4>
```
You are a professional Java test case writer, please create a test case named `supportsBOMinFiles` for the issue `Jsoup-695`, utilizing the provided issue report information and the following function signature.
```java
@Test
public void supportsBOMinFiles() throws IOException {
```
| 103 | [
"org.jsoup.helper.DataUtil"
] | 90198544d0d8ad5a03c93c2afbc24ddb0c57262724c2abd0a1a45c392a73a9bc | @Test
public void supportsBOMinFiles() throws IOException | // You are a professional Java test case writer, please create a test case named `supportsBOMinFiles` for the issue `Jsoup-695`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Jsoup-695
//
// ## Issue-Title:
// UTF16 streams with BOM are processed as UTF-8
//
// ## Issue-Description:
// The handling of the character encoding in org.jsoup.helper.DataUtil.parseByteData(...) is bugged when the input is an UTF16 stream with unicode BOM. This method does a check for presence of a BOM and, if it finds one, incorrectly assumes that this was a UTF-8 BOM. To fix this, the code would have to check the raw BOM bytes as the distinction between the various BOMs is lost after conversion to characters. See also: <http://unicode.org/faq/utf_bom.html#bom4>
//
//
//
//
| Jsoup | package org.jsoup.helper;
import java.io.File;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.parser.Parser;
import org.junit.Test;
import java.nio.ByteBuffer;
import java.nio.charset.Charset;
import static org.jsoup.integration.ParseTest.getFile;
import static org.junit.Assert.*;
public class DataUtilTest {
@Test
public void testCharset() {
assertEquals("utf-8", DataUtil.getCharsetFromContentType("text/html;charset=utf-8 "));
assertEquals("UTF-8", DataUtil.getCharsetFromContentType("text/html; charset=UTF-8"));
assertEquals("ISO-8859-1", DataUtil.getCharsetFromContentType("text/html; charset=ISO-8859-1"));
assertEquals(null, DataUtil.getCharsetFromContentType("text/html"));
assertEquals(null, DataUtil.getCharsetFromContentType(null));
assertEquals(null, DataUtil.getCharsetFromContentType("text/html;charset=Unknown"));
}
@Test public void testQuotedCharset() {
assertEquals("utf-8", DataUtil.getCharsetFromContentType("text/html; charset=\"utf-8\""));
assertEquals("UTF-8", DataUtil.getCharsetFromContentType("text/html;charset=\"UTF-8\""));
assertEquals("ISO-8859-1", DataUtil.getCharsetFromContentType("text/html; charset=\"ISO-8859-1\""));
assertEquals(null, DataUtil.getCharsetFromContentType("text/html; charset=\"Unsupported\""));
assertEquals("UTF-8", DataUtil.getCharsetFromContentType("text/html; charset='UTF-8'"));
}
@Test public void discardsSpuriousByteOrderMark() {
String html = "\uFEFF<html><head><title>One</title></head><body>Two</body></html>";
ByteBuffer buffer = Charset.forName("UTF-8").encode(html);
Document doc = DataUtil.parseByteData(buffer, "UTF-8", "http://foo.com/", Parser.htmlParser());
assertEquals("One", doc.head().text());
}
@Test public void discardsSpuriousByteOrderMarkWhenNoCharsetSet() {
String html = "\uFEFF<html><head><title>One</title></head><body>Two</body></html>";
ByteBuffer buffer = Charset.forName("UTF-8").encode(html);
Document doc = DataUtil.parseByteData(buffer, null, "http://foo.com/", Parser.htmlParser());
assertEquals("One", doc.head().text());
assertEquals("UTF-8", doc.outputSettings().charset().displayName());
}
@Test
public void shouldNotThrowExceptionOnEmptyCharset() {
assertEquals(null, DataUtil.getCharsetFromContentType("text/html; charset="));
assertEquals(null, DataUtil.getCharsetFromContentType("text/html; charset=;"));
}
@Test
public void shouldSelectFirstCharsetOnWeirdMultileCharsetsInMetaTags() {
assertEquals("ISO-8859-1", DataUtil.getCharsetFromContentType("text/html; charset=ISO-8859-1, charset=1251"));
}
@Test
public void shouldCorrectCharsetForDuplicateCharsetString() {
assertEquals("iso-8859-1", DataUtil.getCharsetFromContentType("text/html; charset=charset=iso-8859-1"));
}
@Test
public void shouldReturnNullForIllegalCharsetNames() {
assertEquals(null, DataUtil.getCharsetFromContentType("text/html; charset=$HJKDF§$/("));
}
@Test
public void generatesMimeBoundaries() {
String m1 = DataUtil.mimeBoundary();
String m2 = DataUtil.mimeBoundary();
assertEquals(DataUtil.boundaryLength, m1.length());
assertEquals(DataUtil.boundaryLength, m2.length());
assertNotSame(m1, m2);
}
@Test
public void wrongMetaCharsetFallback() {
try {
final byte[] input = "<html><head><meta charset=iso-8></head><body></body></html>".getBytes("UTF-8");
final ByteBuffer inBuffer = ByteBuffer.wrap(input);
Document doc = DataUtil.parseByteData(inBuffer, null, "http://example.com", Parser.htmlParser());
final String expected = "<html>\n" +
" <head>\n" +
" <meta charset=\"iso-8\">\n" +
" </head>\n" +
" <body></body>\n" +
"</html>";
assertEquals(expected, doc.toString());
} catch( UnsupportedEncodingException ex ) {
fail(ex.getMessage());
}
}
@Test
public void supportsBOMinFiles() throws IOException {
// test files from http://www.i18nl10n.com/korean/utftest/
File in = getFile("/bomtests/bom_utf16be.html");
Document doc = Jsoup.parse(in, null, "http://example.com");
assertTrue(doc.title().contains("UTF-16BE"));
assertTrue(doc.text().contains("가각갂갃간갅"));
in = getFile("/bomtests/bom_utf16le.html");
doc = Jsoup.parse(in, null, "http://example.com");
assertTrue(doc.title().contains("UTF-16LE"));
assertTrue(doc.text().contains("가각갂갃간갅"));
in = getFile("/bomtests/bom_utf32be.html");
doc = Jsoup.parse(in, null, "http://example.com");
assertTrue(doc.title().contains("UTF-32BE"));
assertTrue(doc.text().contains("가각갂갃간갅"));
in = getFile("/bomtests/bom_utf32le.html");
doc = Jsoup.parse(in, null, "http://example.com");
assertTrue(doc.title().contains("UTF-32LE"));
assertTrue(doc.text().contains("가각갂갃간갅"));
}
} |
||
public void testReplace_StringStringArrayStringArray() {
//JAVADOC TESTS START
assertNull(StringUtils.replaceEach(null, new String[]{"a"}, new String[]{"b"}));
assertEquals(StringUtils.replaceEach("", new String[]{"a"}, new String[]{"b"}),"");
assertEquals(StringUtils.replaceEach("aba", null, null),"aba");
assertEquals(StringUtils.replaceEach("aba", new String[0], null),"aba");
assertEquals(StringUtils.replaceEach("aba", null, new String[0]),"aba");
assertEquals(StringUtils.replaceEach("aba", new String[]{"a"}, null),"aba");
assertEquals(StringUtils.replaceEach("aba", new String[]{"a"}, new String[]{""}),"b");
assertEquals(StringUtils.replaceEach("aba", new String[]{null}, new String[]{"a"}),"aba");
assertEquals(StringUtils.replaceEach("abcde", new String[]{"ab", "d"}, new String[]{"w", "t"}),"wcte");
assertEquals(StringUtils.replaceEach("abcde", new String[]{"ab", "d"}, new String[]{"d", "t"}),"dcte");
//JAVADOC TESTS END
assertEquals("bcc", StringUtils.replaceEach("abc", new String[]{"a", "b"}, new String[]{"b", "c"}));
assertEquals("q651.506bera", StringUtils.replaceEach("d216.102oren",
new String[]{"a", "b", "c", "d", "e", "f", "g", "h", "i", "j", "k", "l", "m", "n",
"o", "p", "q", "r", "s", "t", "u", "v", "w", "x", "y", "z", "A", "B", "C", "D",
"E", "F", "G", "H", "I", "J", "K", "L", "M", "N", "O", "P", "Q", "R", "S", "T",
"U", "V", "W", "X", "Y", "Z", "1", "2", "3", "4", "5", "6", "7", "8", "9"},
new String[]{"n", "o", "p", "q", "r", "s", "t", "u", "v", "w", "x", "y", "z", "a",
"b", "c", "d", "e", "f", "g", "h", "i", "j", "k", "l", "m", "N", "O", "P", "Q",
"R", "S", "T", "U", "V", "W", "X", "Y", "Z", "A", "B", "C", "D", "E", "F", "G",
"H", "I", "J", "K", "L", "M", "5", "6", "7", "8", "9", "1", "2", "3", "4"}));
// Test null safety inside arrays - LANG-552
assertEquals(StringUtils.replaceEach("aba", new String[]{"a"}, new String[]{null}),"aba");
assertEquals(StringUtils.replaceEach("aba", new String[]{"a", "b"}, new String[]{"c", null}),"cbc");
} | org.apache.commons.lang3.StringUtilsTest::testReplace_StringStringArrayStringArray | src/test/org/apache/commons/lang3/StringUtilsTest.java | 1,039 | src/test/org/apache/commons/lang3/StringUtilsTest.java | testReplace_StringStringArrayStringArray | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.lang3;
import java.lang.reflect.Constructor;
import java.lang.reflect.Modifier;
import java.util.Arrays;
import java.util.Collections;
import java.util.Iterator;
import java.util.Locale;
import junit.framework.Test;
import junit.framework.TestCase;
import junit.framework.TestSuite;
import junit.textui.TestRunner;
/**
* Unit tests {@link org.apache.commons.lang3.StringUtils}.
*
* @author Apache Software Foundation
* @author Daniel L. Rall
* @author <a href="mailto:[email protected]">Ringo De Smet</a>
* @author <a href="mailto:[email protected]>Fredrik Westermarck</a>
* @author Holger Krauth
* @author <a href="[email protected]">Henning P. Schmiedehausen</a>
* @author Phil Steitz
* @author Gary D. Gregory
* @author Scott Johnson
* @author Al Chou
* @version $Id$
*/
public class StringUtilsTest extends TestCase {
static final String WHITESPACE;
static final String NON_WHITESPACE;
static final String TRIMMABLE;
static final String NON_TRIMMABLE;
static {
String ws = "";
String nws = "";
String tr = "";
String ntr = "";
for (int i = 0; i < Character.MAX_VALUE; i++) {
if (Character.isWhitespace((char) i)) {
ws += String.valueOf((char) i);
if (i > 32) {
ntr += String.valueOf((char) i);
}
} else if (i < 40) {
nws += String.valueOf((char) i);
}
}
for (int i = 0; i <= 32; i++) {
tr += String.valueOf((char) i);
}
WHITESPACE = ws;
NON_WHITESPACE = nws;
TRIMMABLE = tr;
NON_TRIMMABLE = ntr;
}
private static final String[] ARRAY_LIST = { "foo", "bar", "baz" };
private static final String[] EMPTY_ARRAY_LIST = {};
private static final String[] NULL_ARRAY_LIST = {null};
private static final String[] MIXED_ARRAY_LIST = {null, "", "foo"};
private static final Object[] MIXED_TYPE_LIST = {new String("foo"), new Long(2)};
private static final String SEPARATOR = ",";
private static final char SEPARATOR_CHAR = ';';
private static final String TEXT_LIST = "foo,bar,baz";
private static final String TEXT_LIST_CHAR = "foo;bar;baz";
private static final String TEXT_LIST_NOSEP = "foobarbaz";
private static final String FOO_UNCAP = "foo";
private static final String FOO_CAP = "Foo";
private static final String SENTENCE_UNCAP = "foo bar baz";
private static final String SENTENCE_CAP = "Foo Bar Baz";
public StringUtilsTest(String name) {
super(name);
}
public static void main(String[] args) {
TestRunner.run(suite());
}
public static Test suite() {
TestSuite suite = new TestSuite(StringUtilsTest.class);
suite.setName("StringUtilsTest Tests");
return suite;
}
@Override
protected void setUp() throws Exception {
super.setUp();
}
@Override
protected void tearDown() throws Exception {
super.tearDown();
}
//-----------------------------------------------------------------------
public void testConstructor() {
assertNotNull(new StringUtils());
Constructor<?>[] cons = StringUtils.class.getDeclaredConstructors();
assertEquals(1, cons.length);
assertEquals(true, Modifier.isPublic(cons[0].getModifiers()));
assertEquals(true, Modifier.isPublic(StringUtils.class.getModifiers()));
assertEquals(false, Modifier.isFinal(StringUtils.class.getModifiers()));
}
//-----------------------------------------------------------------------
public void testCaseFunctions() {
assertEquals(null, StringUtils.upperCase(null));
assertEquals(null, StringUtils.upperCase(null, Locale.ENGLISH));
assertEquals(null, StringUtils.lowerCase(null));
assertEquals(null, StringUtils.lowerCase(null, Locale.ENGLISH));
assertEquals(null, StringUtils.capitalize(null));
assertEquals(null, StringUtils.uncapitalize(null));
assertEquals("capitalize(empty-string) failed",
"", StringUtils.capitalize("") );
assertEquals("capitalize(single-char-string) failed",
"X", StringUtils.capitalize("x") );
assertEquals("uncapitalize(String) failed",
FOO_UNCAP, StringUtils.uncapitalize(FOO_CAP) );
assertEquals("uncapitalize(empty-string) failed",
"", StringUtils.uncapitalize("") );
assertEquals("uncapitalize(single-char-string) failed",
"x", StringUtils.uncapitalize("X") );
// reflection type of tests: Sentences.
assertEquals("uncapitalize(capitalize(String)) failed",
SENTENCE_UNCAP, StringUtils.uncapitalize(StringUtils.capitalize(SENTENCE_UNCAP)) );
assertEquals("capitalize(uncapitalize(String)) failed",
SENTENCE_CAP, StringUtils.capitalize(StringUtils.uncapitalize(SENTENCE_CAP)) );
// reflection type of tests: One word.
assertEquals("uncapitalize(capitalize(String)) failed",
FOO_UNCAP, StringUtils.uncapitalize(StringUtils.capitalize(FOO_UNCAP)) );
assertEquals("capitalize(uncapitalize(String)) failed",
FOO_CAP, StringUtils.capitalize(StringUtils.uncapitalize(FOO_CAP)) );
assertEquals("upperCase(String) failed",
"FOO TEST THING", StringUtils.upperCase("fOo test THING") );
assertEquals("upperCase(empty-string) failed",
"", StringUtils.upperCase("") );
assertEquals("lowerCase(String) failed",
"foo test thing", StringUtils.lowerCase("fOo test THING") );
assertEquals("lowerCase(empty-string) failed",
"", StringUtils.lowerCase("") );
assertEquals("upperCase(String, Locale) failed",
"FOO TEST THING", StringUtils.upperCase("fOo test THING", Locale.ENGLISH) );
assertEquals("upperCase(empty-string, Locale) failed",
"", StringUtils.upperCase("", Locale.ENGLISH) );
assertEquals("lowerCase(String, Locale) failed",
"foo test thing", StringUtils.lowerCase("fOo test THING", Locale.ENGLISH) );
assertEquals("lowerCase(empty-string, Locale) failed",
"", StringUtils.lowerCase("", Locale.ENGLISH) );
}
public void testSwapCase_String() {
assertEquals(null, StringUtils.swapCase(null));
assertEquals("", StringUtils.swapCase(""));
assertEquals(" ", StringUtils.swapCase(" "));
assertEquals("i", WordUtils.swapCase("I") );
assertEquals("I", WordUtils.swapCase("i") );
assertEquals("I AM HERE 123", StringUtils.swapCase("i am here 123") );
assertEquals("i aM hERE 123", StringUtils.swapCase("I Am Here 123") );
assertEquals("I AM here 123", StringUtils.swapCase("i am HERE 123") );
assertEquals("i am here 123", StringUtils.swapCase("I AM HERE 123") );
String test = "This String contains a TitleCase character: \u01C8";
String expect = "tHIS sTRING CONTAINS A tITLEcASE CHARACTER: \u01C9";
assertEquals(expect, WordUtils.swapCase(test));
}
//-----------------------------------------------------------------------
public void testJoin_Objectarray() {
assertEquals(null, StringUtils.join(null));
assertEquals("", StringUtils.join(EMPTY_ARRAY_LIST));
assertEquals("", StringUtils.join(NULL_ARRAY_LIST));
assertEquals("abc", StringUtils.join(new String[] {"a", "b", "c"}));
assertEquals("a", StringUtils.join(new String[] {null, "a", ""}));
assertEquals("foo", StringUtils.join(MIXED_ARRAY_LIST));
assertEquals("foo2", StringUtils.join(MIXED_TYPE_LIST));
}
public void testJoin_ArrayChar() {
assertEquals(null, StringUtils.join((Object[]) null, ','));
assertEquals(TEXT_LIST_CHAR, StringUtils.join(ARRAY_LIST, SEPARATOR_CHAR));
assertEquals("", StringUtils.join(EMPTY_ARRAY_LIST, SEPARATOR_CHAR));
assertEquals(";;foo", StringUtils.join(MIXED_ARRAY_LIST, SEPARATOR_CHAR));
assertEquals("foo;2", StringUtils.join(MIXED_TYPE_LIST, SEPARATOR_CHAR));
assertEquals("/", StringUtils.join(MIXED_ARRAY_LIST, '/', 0, MIXED_ARRAY_LIST.length-1));
assertEquals("foo", StringUtils.join(MIXED_TYPE_LIST, '/', 0, 1));
assertEquals("foo/2", StringUtils.join(MIXED_TYPE_LIST, '/', 0, 2));
assertEquals("2", StringUtils.join(MIXED_TYPE_LIST, '/', 1, 2));
assertEquals("", StringUtils.join(MIXED_TYPE_LIST, '/', 2, 1));
}
public void testJoin_ArrayString() {
assertEquals(null, StringUtils.join((Object[]) null, null));
assertEquals(TEXT_LIST_NOSEP, StringUtils.join(ARRAY_LIST, null));
assertEquals(TEXT_LIST_NOSEP, StringUtils.join(ARRAY_LIST, ""));
assertEquals("", StringUtils.join(NULL_ARRAY_LIST, null));
assertEquals("", StringUtils.join(EMPTY_ARRAY_LIST, null));
assertEquals("", StringUtils.join(EMPTY_ARRAY_LIST, ""));
assertEquals("", StringUtils.join(EMPTY_ARRAY_LIST, SEPARATOR));
assertEquals(TEXT_LIST, StringUtils.join(ARRAY_LIST, SEPARATOR));
assertEquals(",,foo", StringUtils.join(MIXED_ARRAY_LIST, SEPARATOR));
assertEquals("foo,2", StringUtils.join(MIXED_TYPE_LIST, SEPARATOR));
assertEquals("/", StringUtils.join(MIXED_ARRAY_LIST, "/", 0, MIXED_ARRAY_LIST.length-1));
assertEquals("", StringUtils.join(MIXED_ARRAY_LIST, "", 0, MIXED_ARRAY_LIST.length-1));
assertEquals("foo", StringUtils.join(MIXED_TYPE_LIST, "/", 0, 1));
assertEquals("foo/2", StringUtils.join(MIXED_TYPE_LIST, "/", 0, 2));
assertEquals("2", StringUtils.join(MIXED_TYPE_LIST, "/", 1, 2));
assertEquals("", StringUtils.join(MIXED_TYPE_LIST, "/", 2, 1));
}
public void testJoin_IteratorChar() {
assertEquals(null, StringUtils.join((Iterator<?>) null, ','));
assertEquals(TEXT_LIST_CHAR, StringUtils.join(Arrays.asList(ARRAY_LIST).iterator(), SEPARATOR_CHAR));
assertEquals("", StringUtils.join(Arrays.asList(NULL_ARRAY_LIST).iterator(), SEPARATOR_CHAR));
assertEquals("", StringUtils.join(Arrays.asList(EMPTY_ARRAY_LIST).iterator(), SEPARATOR_CHAR));
assertEquals("foo", StringUtils.join(Collections.singleton("foo").iterator(), 'x'));
}
public void testJoin_IteratorString() {
assertEquals(null, StringUtils.join((Iterator<?>) null, null));
assertEquals(TEXT_LIST_NOSEP, StringUtils.join(Arrays.asList(ARRAY_LIST).iterator(), null));
assertEquals(TEXT_LIST_NOSEP, StringUtils.join(Arrays.asList(ARRAY_LIST).iterator(), ""));
assertEquals("foo", StringUtils.join(Collections.singleton("foo").iterator(), "x"));
assertEquals("foo", StringUtils.join(Collections.singleton("foo").iterator(), null));
assertEquals("", StringUtils.join(Arrays.asList(NULL_ARRAY_LIST).iterator(), null));
assertEquals("", StringUtils.join(Arrays.asList(EMPTY_ARRAY_LIST).iterator(), null));
assertEquals("", StringUtils.join(Arrays.asList(EMPTY_ARRAY_LIST).iterator(), ""));
assertEquals("", StringUtils.join(Arrays.asList(EMPTY_ARRAY_LIST).iterator(), SEPARATOR));
assertEquals(TEXT_LIST, StringUtils.join(Arrays.asList(ARRAY_LIST).iterator(), SEPARATOR));
}
public void testJoin_IterableChar() {
assertEquals(null, StringUtils.join((Iterable<?>) null, ','));
assertEquals(TEXT_LIST_CHAR, StringUtils.join(Arrays.asList(ARRAY_LIST), SEPARATOR_CHAR));
assertEquals("", StringUtils.join(Arrays.asList(NULL_ARRAY_LIST), SEPARATOR_CHAR));
assertEquals("", StringUtils.join(Arrays.asList(EMPTY_ARRAY_LIST), SEPARATOR_CHAR));
assertEquals("foo", StringUtils.join(Collections.singleton("foo"), 'x'));
}
public void testJoin_IterableString() {
assertEquals(null, StringUtils.join((Iterable<?>) null, null));
assertEquals(TEXT_LIST_NOSEP, StringUtils.join(Arrays.asList(ARRAY_LIST), null));
assertEquals(TEXT_LIST_NOSEP, StringUtils.join(Arrays.asList(ARRAY_LIST), ""));
assertEquals("foo", StringUtils.join(Collections.singleton("foo"), "x"));
assertEquals("foo", StringUtils.join(Collections.singleton("foo"), null));
assertEquals("", StringUtils.join(Arrays.asList(NULL_ARRAY_LIST), null));
assertEquals("", StringUtils.join(Arrays.asList(EMPTY_ARRAY_LIST), null));
assertEquals("", StringUtils.join(Arrays.asList(EMPTY_ARRAY_LIST), ""));
assertEquals("", StringUtils.join(Arrays.asList(EMPTY_ARRAY_LIST), SEPARATOR));
assertEquals(TEXT_LIST, StringUtils.join(Arrays.asList(ARRAY_LIST), SEPARATOR));
}
public void testSplit_String() {
assertEquals(null, StringUtils.split(null));
assertEquals(0, StringUtils.split("").length);
String str = "a b .c";
String[] res = StringUtils.split(str);
assertEquals(3, res.length);
assertEquals("a", res[0]);
assertEquals("b", res[1]);
assertEquals(".c", res[2]);
str = " a ";
res = StringUtils.split(str);
assertEquals(1, res.length);
assertEquals("a", res[0]);
str = "a" + WHITESPACE + "b" + NON_WHITESPACE + "c";
res = StringUtils.split(str);
assertEquals(2, res.length);
assertEquals("a", res[0]);
assertEquals("b" + NON_WHITESPACE + "c", res[1]);
}
public void testSplit_StringChar() {
assertEquals(null, StringUtils.split(null, '.'));
assertEquals(0, StringUtils.split("", '.').length);
String str = "a.b.. c";
String[] res = StringUtils.split(str, '.');
assertEquals(3, res.length);
assertEquals("a", res[0]);
assertEquals("b", res[1]);
assertEquals(" c", res[2]);
str = ".a.";
res = StringUtils.split(str, '.');
assertEquals(1, res.length);
assertEquals("a", res[0]);
str = "a b c";
res = StringUtils.split(str,' ');
assertEquals(3, res.length);
assertEquals("a", res[0]);
assertEquals("b", res[1]);
assertEquals("c", res[2]);
}
public void testSplit_StringString_StringStringInt() {
assertEquals(null, StringUtils.split(null, "."));
assertEquals(null, StringUtils.split(null, ".", 3));
assertEquals(0, StringUtils.split("", ".").length);
assertEquals(0, StringUtils.split("", ".", 3).length);
innerTestSplit('.', ".", ' ');
innerTestSplit('.', ".", ',');
innerTestSplit('.', ".,", 'x');
for (int i = 0; i < WHITESPACE.length(); i++) {
for (int j = 0; j < NON_WHITESPACE.length(); j++) {
innerTestSplit(WHITESPACE.charAt(i), null, NON_WHITESPACE.charAt(j));
innerTestSplit(WHITESPACE.charAt(i), String.valueOf(WHITESPACE.charAt(i)), NON_WHITESPACE.charAt(j));
}
}
String[] results = null;
String[] expectedResults = {"ab", "de fg"};
results = StringUtils.split("ab de fg", null, 2);
assertEquals(expectedResults.length, results.length);
for (int i = 0; i < expectedResults.length; i++) {
assertEquals(expectedResults[i], results[i]);
}
String[] expectedResults2 = {"ab", "cd:ef"};
results = StringUtils.split("ab:cd:ef",":", 2);
assertEquals(expectedResults2.length, results.length);
for (int i = 0; i < expectedResults2.length; i++) {
assertEquals(expectedResults2[i], results[i]);
}
}
private void innerTestSplit(char separator, String sepStr, char noMatch) {
String msg = "Failed on separator hex(" + Integer.toHexString(separator) +
"), noMatch hex(" + Integer.toHexString(noMatch) + "), sepStr(" + sepStr + ")";
final String str = "a" + separator + "b" + separator + separator + noMatch + "c";
String[] res;
// (str, sepStr)
res = StringUtils.split(str, sepStr);
assertEquals(msg, 3, res.length);
assertEquals(msg, "a", res[0]);
assertEquals(msg, "b", res[1]);
assertEquals(msg, noMatch + "c", res[2]);
final String str2 = separator + "a" + separator;
res = StringUtils.split(str2, sepStr);
assertEquals(msg, 1, res.length);
assertEquals(msg, "a", res[0]);
res = StringUtils.split(str, sepStr, -1);
assertEquals(msg, 3, res.length);
assertEquals(msg, "a", res[0]);
assertEquals(msg, "b", res[1]);
assertEquals(msg, noMatch + "c", res[2]);
res = StringUtils.split(str, sepStr, 0);
assertEquals(msg, 3, res.length);
assertEquals(msg, "a", res[0]);
assertEquals(msg, "b", res[1]);
assertEquals(msg, noMatch + "c", res[2]);
res = StringUtils.split(str, sepStr, 1);
assertEquals(msg, 1, res.length);
assertEquals(msg, str, res[0]);
res = StringUtils.split(str, sepStr, 2);
assertEquals(msg, 2, res.length);
assertEquals(msg, "a", res[0]);
assertEquals(msg, str.substring(2), res[1]);
}
public void testSplitByWholeString_StringStringBoolean() {
assertEquals( null, StringUtils.splitByWholeSeparator( null, "." ) ) ;
assertEquals( 0, StringUtils.splitByWholeSeparator( "", "." ).length ) ;
String stringToSplitOnNulls = "ab de fg" ;
String[] splitOnNullExpectedResults = { "ab", "de", "fg" } ;
String[] splitOnNullResults = StringUtils.splitByWholeSeparator( stringToSplitOnNulls, null ) ;
assertEquals( splitOnNullExpectedResults.length, splitOnNullResults.length ) ;
for ( int i = 0 ; i < splitOnNullExpectedResults.length ; i+= 1 ) {
assertEquals( splitOnNullExpectedResults[i], splitOnNullResults[i] ) ;
}
String stringToSplitOnCharactersAndString = "abstemiouslyaeiouyabstemiously" ;
String[] splitOnStringExpectedResults = { "abstemiously", "abstemiously" } ;
String[] splitOnStringResults = StringUtils.splitByWholeSeparator( stringToSplitOnCharactersAndString, "aeiouy" ) ;
assertEquals( splitOnStringExpectedResults.length, splitOnStringResults.length ) ;
for ( int i = 0 ; i < splitOnStringExpectedResults.length ; i+= 1 ) {
assertEquals( splitOnStringExpectedResults[i], splitOnStringResults[i] ) ;
}
String[] splitWithMultipleSeparatorExpectedResults = {"ab", "cd", "ef"};
String[] splitWithMultipleSeparator = StringUtils.splitByWholeSeparator("ab:cd::ef", ":");
assertEquals( splitWithMultipleSeparatorExpectedResults.length, splitWithMultipleSeparator.length );
for( int i = 0; i < splitWithMultipleSeparatorExpectedResults.length ; i++ ) {
assertEquals( splitWithMultipleSeparatorExpectedResults[i], splitWithMultipleSeparator[i] ) ;
}
}
public void testSplitByWholeString_StringStringBooleanInt() {
assertEquals( null, StringUtils.splitByWholeSeparator( null, ".", 3 ) ) ;
assertEquals( 0, StringUtils.splitByWholeSeparator( "", ".", 3 ).length ) ;
String stringToSplitOnNulls = "ab de fg" ;
String[] splitOnNullExpectedResults = { "ab", "de fg" } ;
//String[] splitOnNullExpectedResults = { "ab", "de" } ;
String[] splitOnNullResults = StringUtils.splitByWholeSeparator( stringToSplitOnNulls, null, 2 ) ;
assertEquals( splitOnNullExpectedResults.length, splitOnNullResults.length ) ;
for ( int i = 0 ; i < splitOnNullExpectedResults.length ; i+= 1 ) {
assertEquals( splitOnNullExpectedResults[i], splitOnNullResults[i] ) ;
}
String stringToSplitOnCharactersAndString = "abstemiouslyaeiouyabstemiouslyaeiouyabstemiously" ;
String[] splitOnStringExpectedResults = { "abstemiously", "abstemiouslyaeiouyabstemiously" } ;
//String[] splitOnStringExpectedResults = { "abstemiously", "abstemiously" } ;
String[] splitOnStringResults = StringUtils.splitByWholeSeparator( stringToSplitOnCharactersAndString, "aeiouy", 2 ) ;
assertEquals( splitOnStringExpectedResults.length, splitOnStringResults.length ) ;
for ( int i = 0 ; i < splitOnStringExpectedResults.length ; i++ ) {
assertEquals( splitOnStringExpectedResults[i], splitOnStringResults[i] ) ;
}
}
public void testSplitByWholeSeparatorPreserveAllTokens_StringStringInt() {
assertEquals( null, StringUtils.splitByWholeSeparatorPreserveAllTokens( null, ".", -1 ) ) ;
assertEquals( 0, StringUtils.splitByWholeSeparatorPreserveAllTokens( "", ".", -1 ).length ) ;
// test whitespace
String input = "ab de fg" ;
String[] expected = new String[] { "ab", "", "", "de", "fg" } ;
String[] actual = StringUtils.splitByWholeSeparatorPreserveAllTokens( input, null, -1 ) ;
assertEquals( expected.length, actual.length ) ;
for ( int i = 0 ; i < actual.length ; i+= 1 ) {
assertEquals( expected[i], actual[i] );
}
// test delimiter singlechar
input = "1::2:::3::::4";
expected = new String[] { "1", "", "2", "", "", "3", "", "", "", "4" };
actual = StringUtils.splitByWholeSeparatorPreserveAllTokens( input, ":", -1 ) ;
assertEquals( expected.length, actual.length ) ;
for ( int i = 0 ; i < actual.length ; i+= 1 ) {
assertEquals( expected[i], actual[i] );
}
// test delimiter multichar
input = "1::2:::3::::4";
expected = new String[] { "1", "2", ":3", "", "4" };
actual = StringUtils.splitByWholeSeparatorPreserveAllTokens( input, "::", -1 ) ;
assertEquals( expected.length, actual.length ) ;
for ( int i = 0 ; i < actual.length ; i+= 1 ) {
assertEquals( expected[i], actual[i] );
}
// test delimiter char with max
input = "1::2::3:4";
expected = new String[] { "1", "", "2", ":3:4" };
actual = StringUtils.splitByWholeSeparatorPreserveAllTokens( input, ":", 4 ) ;
assertEquals( expected.length, actual.length ) ;
for ( int i = 0 ; i < actual.length ; i+= 1 ) {
assertEquals( expected[i], actual[i] );
}
}
public void testSplitPreserveAllTokens_String() {
assertEquals(null, StringUtils.splitPreserveAllTokens(null));
assertEquals(0, StringUtils.splitPreserveAllTokens("").length);
String str = "abc def";
String[] res = StringUtils.splitPreserveAllTokens(str);
assertEquals(2, res.length);
assertEquals("abc", res[0]);
assertEquals("def", res[1]);
str = "abc def";
res = StringUtils.splitPreserveAllTokens(str);
assertEquals(3, res.length);
assertEquals("abc", res[0]);
assertEquals("", res[1]);
assertEquals("def", res[2]);
str = " abc ";
res = StringUtils.splitPreserveAllTokens(str);
assertEquals(3, res.length);
assertEquals("", res[0]);
assertEquals("abc", res[1]);
assertEquals("", res[2]);
str = "a b .c";
res = StringUtils.splitPreserveAllTokens(str);
assertEquals(3, res.length);
assertEquals("a", res[0]);
assertEquals("b", res[1]);
assertEquals(".c", res[2]);
str = " a b .c";
res = StringUtils.splitPreserveAllTokens(str);
assertEquals(4, res.length);
assertEquals("", res[0]);
assertEquals("a", res[1]);
assertEquals("b", res[2]);
assertEquals(".c", res[3]);
str = "a b .c";
res = StringUtils.splitPreserveAllTokens(str);
assertEquals(5, res.length);
assertEquals("a", res[0]);
assertEquals("", res[1]);
assertEquals("b", res[2]);
assertEquals("", res[3]);
assertEquals(".c", res[4]);
str = " a ";
res = StringUtils.splitPreserveAllTokens(str);
assertEquals(4, res.length);
assertEquals("", res[0]);
assertEquals("a", res[1]);
assertEquals("", res[2]);
assertEquals("", res[3]);
str = " a b";
res = StringUtils.splitPreserveAllTokens(str);
assertEquals(4, res.length);
assertEquals("", res[0]);
assertEquals("a", res[1]);
assertEquals("", res[2]);
assertEquals("b", res[3]);
str = "a" + WHITESPACE + "b" + NON_WHITESPACE + "c";
res = StringUtils.splitPreserveAllTokens(str);
assertEquals(WHITESPACE.length() + 1, res.length);
assertEquals("a", res[0]);
for(int i = 1; i < WHITESPACE.length()-1; i++)
{
assertEquals("", res[i]);
}
assertEquals("b" + NON_WHITESPACE + "c", res[WHITESPACE.length()]);
}
public void testSplitPreserveAllTokens_StringChar() {
assertEquals(null, StringUtils.splitPreserveAllTokens(null, '.'));
assertEquals(0, StringUtils.splitPreserveAllTokens("", '.').length);
String str = "a.b. c";
String[] res = StringUtils.splitPreserveAllTokens(str, '.');
assertEquals(3, res.length);
assertEquals("a", res[0]);
assertEquals("b", res[1]);
assertEquals(" c", res[2]);
str = "a.b.. c";
res = StringUtils.splitPreserveAllTokens(str, '.');
assertEquals(4, res.length);
assertEquals("a", res[0]);
assertEquals("b", res[1]);
assertEquals("", res[2]);
assertEquals(" c", res[3]);
str = ".a.";
res = StringUtils.splitPreserveAllTokens(str, '.');
assertEquals(3, res.length);
assertEquals("", res[0]);
assertEquals("a", res[1]);
assertEquals("", res[2]);
str = ".a..";
res = StringUtils.splitPreserveAllTokens(str, '.');
assertEquals(4, res.length);
assertEquals("", res[0]);
assertEquals("a", res[1]);
assertEquals("", res[2]);
assertEquals("", res[3]);
str = "..a.";
res = StringUtils.splitPreserveAllTokens(str, '.');
assertEquals(4, res.length);
assertEquals("", res[0]);
assertEquals("", res[1]);
assertEquals("a", res[2]);
assertEquals("", res[3]);
str = "..a";
res = StringUtils.splitPreserveAllTokens(str, '.');
assertEquals(3, res.length);
assertEquals("", res[0]);
assertEquals("", res[1]);
assertEquals("a", res[2]);
str = "a b c";
res = StringUtils.splitPreserveAllTokens(str,' ');
assertEquals(3, res.length);
assertEquals("a", res[0]);
assertEquals("b", res[1]);
assertEquals("c", res[2]);
str = "a b c";
res = StringUtils.splitPreserveAllTokens(str,' ');
assertEquals(5, res.length);
assertEquals("a", res[0]);
assertEquals("", res[1]);
assertEquals("b", res[2]);
assertEquals("", res[3]);
assertEquals("c", res[4]);
str = " a b c";
res = StringUtils.splitPreserveAllTokens(str,' ');
assertEquals(4, res.length);
assertEquals("", res[0]);
assertEquals("a", res[1]);
assertEquals("b", res[2]);
assertEquals("c", res[3]);
str = " a b c";
res = StringUtils.splitPreserveAllTokens(str,' ');
assertEquals(5, res.length);
assertEquals("", res[0]);
assertEquals("", res[1]);
assertEquals("a", res[2]);
assertEquals("b", res[3]);
assertEquals("c", res[4]);
str = "a b c ";
res = StringUtils.splitPreserveAllTokens(str,' ');
assertEquals(4, res.length);
assertEquals("a", res[0]);
assertEquals("b", res[1]);
assertEquals("c", res[2]);
assertEquals("", res[3]);
str = "a b c ";
res = StringUtils.splitPreserveAllTokens(str,' ');
assertEquals(5, res.length);
assertEquals("a", res[0]);
assertEquals("b", res[1]);
assertEquals("c", res[2]);
assertEquals("", res[3]);
assertEquals("", res[3]);
// Match example in javadoc
{
String[] results = null;
String[] expectedResults = {"a", "", "b", "c"};
results = StringUtils.splitPreserveAllTokens("a..b.c",'.');
assertEquals(expectedResults.length, results.length);
for (int i = 0; i < expectedResults.length; i++) {
assertEquals(expectedResults[i], results[i]);
}
}
}
public void testSplitPreserveAllTokens_StringString_StringStringInt() {
assertEquals(null, StringUtils.splitPreserveAllTokens(null, "."));
assertEquals(null, StringUtils.splitPreserveAllTokens(null, ".", 3));
assertEquals(0, StringUtils.splitPreserveAllTokens("", ".").length);
assertEquals(0, StringUtils.splitPreserveAllTokens("", ".", 3).length);
innerTestSplitPreserveAllTokens('.', ".", ' ');
innerTestSplitPreserveAllTokens('.', ".", ',');
innerTestSplitPreserveAllTokens('.', ".,", 'x');
for (int i = 0; i < WHITESPACE.length(); i++) {
for (int j = 0; j < NON_WHITESPACE.length(); j++) {
innerTestSplitPreserveAllTokens(WHITESPACE.charAt(i), null, NON_WHITESPACE.charAt(j));
innerTestSplitPreserveAllTokens(WHITESPACE.charAt(i), String.valueOf(WHITESPACE.charAt(i)), NON_WHITESPACE.charAt(j));
}
}
{
String[] results = null;
String[] expectedResults = {"ab", "de fg"};
results = StringUtils.splitPreserveAllTokens("ab de fg", null, 2);
assertEquals(expectedResults.length, results.length);
for (int i = 0; i < expectedResults.length; i++) {
assertEquals(expectedResults[i], results[i]);
}
}
{
String[] results = null;
String[] expectedResults = {"ab", " de fg"};
results = StringUtils.splitPreserveAllTokens("ab de fg", null, 2);
assertEquals(expectedResults.length, results.length);
for (int i = 0; i < expectedResults.length; i++) {
assertEquals(expectedResults[i], results[i]);
}
}
{
String[] results = null;
String[] expectedResults = {"ab", "::de:fg"};
results = StringUtils.splitPreserveAllTokens("ab:::de:fg", ":", 2);
assertEquals(expectedResults.length, results.length);
for (int i = 0; i < expectedResults.length; i++) {
assertEquals(expectedResults[i], results[i]);
}
}
{
String[] results = null;
String[] expectedResults = {"ab", "", " de fg"};
results = StringUtils.splitPreserveAllTokens("ab de fg", null, 3);
assertEquals(expectedResults.length, results.length);
for (int i = 0; i < expectedResults.length; i++) {
assertEquals(expectedResults[i], results[i]);
}
}
{
String[] results = null;
String[] expectedResults = {"ab", "", "", "de fg"};
results = StringUtils.splitPreserveAllTokens("ab de fg", null, 4);
assertEquals(expectedResults.length, results.length);
for (int i = 0; i < expectedResults.length; i++) {
assertEquals(expectedResults[i], results[i]);
}
}
{
String[] expectedResults = {"ab", "cd:ef"};
String[] results = null;
results = StringUtils.splitPreserveAllTokens("ab:cd:ef",":", 2);
assertEquals(expectedResults.length, results.length);
for (int i = 0; i < expectedResults.length; i++) {
assertEquals(expectedResults[i], results[i]);
}
}
{
String[] results = null;
String[] expectedResults = {"ab", ":cd:ef"};
results = StringUtils.splitPreserveAllTokens("ab::cd:ef",":", 2);
assertEquals(expectedResults.length, results.length);
for (int i = 0; i < expectedResults.length; i++) {
assertEquals(expectedResults[i], results[i]);
}
}
{
String[] results = null;
String[] expectedResults = {"ab", "", ":cd:ef"};
results = StringUtils.splitPreserveAllTokens("ab:::cd:ef",":", 3);
assertEquals(expectedResults.length, results.length);
for (int i = 0; i < expectedResults.length; i++) {
assertEquals(expectedResults[i], results[i]);
}
}
{
String[] results = null;
String[] expectedResults = {"ab", "", "", "cd:ef"};
results = StringUtils.splitPreserveAllTokens("ab:::cd:ef",":", 4);
assertEquals(expectedResults.length, results.length);
for (int i = 0; i < expectedResults.length; i++) {
assertEquals(expectedResults[i], results[i]);
}
}
{
String[] results = null;
String[] expectedResults = {"", "ab", "", "", "cd:ef"};
results = StringUtils.splitPreserveAllTokens(":ab:::cd:ef",":", 5);
assertEquals(expectedResults.length, results.length);
for (int i = 0; i < expectedResults.length; i++) {
assertEquals(expectedResults[i], results[i]);
}
}
{
String[] results = null;
String[] expectedResults = {"", "", "ab", "", "", "cd:ef"};
results = StringUtils.splitPreserveAllTokens("::ab:::cd:ef",":", 6);
assertEquals(expectedResults.length, results.length);
for (int i = 0; i < expectedResults.length; i++) {
assertEquals(expectedResults[i], results[i]);
}
}
}
private void innerTestSplitPreserveAllTokens(char separator, String sepStr, char noMatch) {
String msg = "Failed on separator hex(" + Integer.toHexString(separator) +
"), noMatch hex(" + Integer.toHexString(noMatch) + "), sepStr(" + sepStr + ")";
final String str = "a" + separator + "b" + separator + separator + noMatch + "c";
String[] res;
// (str, sepStr)
res = StringUtils.splitPreserveAllTokens(str, sepStr);
assertEquals(msg, 4, res.length);
assertEquals(msg, "a", res[0]);
assertEquals(msg, "b", res[1]);
assertEquals(msg, "", res[2]);
assertEquals(msg, noMatch + "c", res[3]);
final String str2 = separator + "a" + separator;
res = StringUtils.splitPreserveAllTokens(str2, sepStr);
assertEquals(msg, 3, res.length);
assertEquals(msg, "", res[0]);
assertEquals(msg, "a", res[1]);
assertEquals(msg, "", res[2]);
res = StringUtils.splitPreserveAllTokens(str, sepStr, -1);
assertEquals(msg, 4, res.length);
assertEquals(msg, "a", res[0]);
assertEquals(msg, "b", res[1]);
assertEquals(msg, "", res[2]);
assertEquals(msg, noMatch + "c", res[3]);
res = StringUtils.splitPreserveAllTokens(str, sepStr, 0);
assertEquals(msg, 4, res.length);
assertEquals(msg, "a", res[0]);
assertEquals(msg, "b", res[1]);
assertEquals(msg, "", res[2]);
assertEquals(msg, noMatch + "c", res[3]);
res = StringUtils.splitPreserveAllTokens(str, sepStr, 1);
assertEquals(msg, 1, res.length);
assertEquals(msg, str, res[0]);
res = StringUtils.splitPreserveAllTokens(str, sepStr, 2);
assertEquals(msg, 2, res.length);
assertEquals(msg, "a", res[0]);
assertEquals(msg, str.substring(2), res[1]);
}
public void testSplitByCharacterType() {
assertNull(StringUtils.splitByCharacterType(null));
assertEquals(0, StringUtils.splitByCharacterType("").length);
assertTrue(ArrayUtils.isEquals(new String[] { "ab", " ", "de", " ",
"fg" }, StringUtils.splitByCharacterType("ab de fg")));
assertTrue(ArrayUtils.isEquals(new String[] { "ab", " ", "de", " ",
"fg" }, StringUtils.splitByCharacterType("ab de fg")));
assertTrue(ArrayUtils.isEquals(new String[] { "ab", ":", "cd", ":",
"ef" }, StringUtils.splitByCharacterType("ab:cd:ef")));
assertTrue(ArrayUtils.isEquals(new String[] { "number", "5" },
StringUtils.splitByCharacterType("number5")));
assertTrue(ArrayUtils.isEquals(new String[] { "foo", "B", "ar" },
StringUtils.splitByCharacterType("fooBar")));
assertTrue(ArrayUtils.isEquals(new String[] { "foo", "200", "B", "ar" },
StringUtils.splitByCharacterType("foo200Bar")));
assertTrue(ArrayUtils.isEquals(new String[] { "ASFR", "ules" },
StringUtils.splitByCharacterType("ASFRules")));
}
public void testSplitByCharacterTypeCamelCase() {
assertNull(StringUtils.splitByCharacterTypeCamelCase(null));
assertEquals(0, StringUtils.splitByCharacterTypeCamelCase("").length);
assertTrue(ArrayUtils.isEquals(new String[] { "ab", " ", "de", " ",
"fg" }, StringUtils.splitByCharacterTypeCamelCase("ab de fg")));
assertTrue(ArrayUtils.isEquals(new String[] { "ab", " ", "de", " ",
"fg" }, StringUtils.splitByCharacterTypeCamelCase("ab de fg")));
assertTrue(ArrayUtils.isEquals(new String[] { "ab", ":", "cd", ":",
"ef" }, StringUtils.splitByCharacterTypeCamelCase("ab:cd:ef")));
assertTrue(ArrayUtils.isEquals(new String[] { "number", "5" },
StringUtils.splitByCharacterTypeCamelCase("number5")));
assertTrue(ArrayUtils.isEquals(new String[] { "foo", "Bar" },
StringUtils.splitByCharacterTypeCamelCase("fooBar")));
assertTrue(ArrayUtils.isEquals(new String[] { "foo", "200", "Bar" },
StringUtils.splitByCharacterTypeCamelCase("foo200Bar")));
assertTrue(ArrayUtils.isEquals(new String[] { "ASF", "Rules" },
StringUtils.splitByCharacterTypeCamelCase("ASFRules")));
}
public void testDeleteWhitespace_String() {
assertEquals(null, StringUtils.deleteWhitespace(null));
assertEquals("", StringUtils.deleteWhitespace(""));
assertEquals("", StringUtils.deleteWhitespace(" \u000C \t\t\u001F\n\n \u000B "));
assertEquals("", StringUtils.deleteWhitespace(StringUtilsTest.WHITESPACE));
assertEquals(StringUtilsTest.NON_WHITESPACE, StringUtils.deleteWhitespace(StringUtilsTest.NON_WHITESPACE));
// Note: u-2007 and u-000A both cause problems in the source code
// it should ignore 2007 but delete 000A
assertEquals("\u00A0\u202F", StringUtils.deleteWhitespace(" \u00A0 \t\t\n\n \u202F "));
assertEquals("\u00A0\u202F", StringUtils.deleteWhitespace("\u00A0\u202F"));
assertEquals("test", StringUtils.deleteWhitespace("\u000Bt \t\n\u0009e\rs\n\n \tt"));
}
public void testReplace_StringStringString() {
assertEquals(null, StringUtils.replace(null, null, null));
assertEquals(null, StringUtils.replace(null, null, "any"));
assertEquals(null, StringUtils.replace(null, "any", null));
assertEquals(null, StringUtils.replace(null, "any", "any"));
assertEquals("", StringUtils.replace("", null, null));
assertEquals("", StringUtils.replace("", null, "any"));
assertEquals("", StringUtils.replace("", "any", null));
assertEquals("", StringUtils.replace("", "any", "any"));
assertEquals("FOO", StringUtils.replace("FOO", "", "any"));
assertEquals("FOO", StringUtils.replace("FOO", null, "any"));
assertEquals("FOO", StringUtils.replace("FOO", "F", null));
assertEquals("FOO", StringUtils.replace("FOO", null, null));
assertEquals("", StringUtils.replace("foofoofoo", "foo", ""));
assertEquals("barbarbar", StringUtils.replace("foofoofoo", "foo", "bar"));
assertEquals("farfarfar", StringUtils.replace("foofoofoo", "oo", "ar"));
}
public void testReplace_StringStringStringInt() {
assertEquals(null, StringUtils.replace(null, null, null, 2));
assertEquals(null, StringUtils.replace(null, null, "any", 2));
assertEquals(null, StringUtils.replace(null, "any", null, 2));
assertEquals(null, StringUtils.replace(null, "any", "any", 2));
assertEquals("", StringUtils.replace("", null, null, 2));
assertEquals("", StringUtils.replace("", null, "any", 2));
assertEquals("", StringUtils.replace("", "any", null, 2));
assertEquals("", StringUtils.replace("", "any", "any", 2));
String str = new String(new char[] {'o', 'o', 'f', 'o', 'o'});
assertSame(str, StringUtils.replace(str, "x", "", -1));
assertEquals("f", StringUtils.replace("oofoo", "o", "", -1));
assertEquals("oofoo", StringUtils.replace("oofoo", "o", "", 0));
assertEquals("ofoo", StringUtils.replace("oofoo", "o", "", 1));
assertEquals("foo", StringUtils.replace("oofoo", "o", "", 2));
assertEquals("fo", StringUtils.replace("oofoo", "o", "", 3));
assertEquals("f", StringUtils.replace("oofoo", "o", "", 4));
assertEquals("f", StringUtils.replace("oofoo", "o", "", -5));
assertEquals("f", StringUtils.replace("oofoo", "o", "", 1000));
}
public void testReplaceOnce_StringStringString() {
assertEquals(null, StringUtils.replaceOnce(null, null, null));
assertEquals(null, StringUtils.replaceOnce(null, null, "any"));
assertEquals(null, StringUtils.replaceOnce(null, "any", null));
assertEquals(null, StringUtils.replaceOnce(null, "any", "any"));
assertEquals("", StringUtils.replaceOnce("", null, null));
assertEquals("", StringUtils.replaceOnce("", null, "any"));
assertEquals("", StringUtils.replaceOnce("", "any", null));
assertEquals("", StringUtils.replaceOnce("", "any", "any"));
assertEquals("FOO", StringUtils.replaceOnce("FOO", "", "any"));
assertEquals("FOO", StringUtils.replaceOnce("FOO", null, "any"));
assertEquals("FOO", StringUtils.replaceOnce("FOO", "F", null));
assertEquals("FOO", StringUtils.replaceOnce("FOO", null, null));
assertEquals("foofoo", StringUtils.replaceOnce("foofoofoo", "foo", ""));
}
/**
* Test method for 'StringUtils.replaceEach(String, String[], String[])'
*/
public void testReplace_StringStringArrayStringArray() {
//JAVADOC TESTS START
assertNull(StringUtils.replaceEach(null, new String[]{"a"}, new String[]{"b"}));
assertEquals(StringUtils.replaceEach("", new String[]{"a"}, new String[]{"b"}),"");
assertEquals(StringUtils.replaceEach("aba", null, null),"aba");
assertEquals(StringUtils.replaceEach("aba", new String[0], null),"aba");
assertEquals(StringUtils.replaceEach("aba", null, new String[0]),"aba");
assertEquals(StringUtils.replaceEach("aba", new String[]{"a"}, null),"aba");
assertEquals(StringUtils.replaceEach("aba", new String[]{"a"}, new String[]{""}),"b");
assertEquals(StringUtils.replaceEach("aba", new String[]{null}, new String[]{"a"}),"aba");
assertEquals(StringUtils.replaceEach("abcde", new String[]{"ab", "d"}, new String[]{"w", "t"}),"wcte");
assertEquals(StringUtils.replaceEach("abcde", new String[]{"ab", "d"}, new String[]{"d", "t"}),"dcte");
//JAVADOC TESTS END
assertEquals("bcc", StringUtils.replaceEach("abc", new String[]{"a", "b"}, new String[]{"b", "c"}));
assertEquals("q651.506bera", StringUtils.replaceEach("d216.102oren",
new String[]{"a", "b", "c", "d", "e", "f", "g", "h", "i", "j", "k", "l", "m", "n",
"o", "p", "q", "r", "s", "t", "u", "v", "w", "x", "y", "z", "A", "B", "C", "D",
"E", "F", "G", "H", "I", "J", "K", "L", "M", "N", "O", "P", "Q", "R", "S", "T",
"U", "V", "W", "X", "Y", "Z", "1", "2", "3", "4", "5", "6", "7", "8", "9"},
new String[]{"n", "o", "p", "q", "r", "s", "t", "u", "v", "w", "x", "y", "z", "a",
"b", "c", "d", "e", "f", "g", "h", "i", "j", "k", "l", "m", "N", "O", "P", "Q",
"R", "S", "T", "U", "V", "W", "X", "Y", "Z", "A", "B", "C", "D", "E", "F", "G",
"H", "I", "J", "K", "L", "M", "5", "6", "7", "8", "9", "1", "2", "3", "4"}));
// Test null safety inside arrays - LANG-552
assertEquals(StringUtils.replaceEach("aba", new String[]{"a"}, new String[]{null}),"aba");
assertEquals(StringUtils.replaceEach("aba", new String[]{"a", "b"}, new String[]{"c", null}),"cbc");
}
/**
* Test method for 'StringUtils.replaceEachRepeatedly(String, String[], String[])'
*/
public void testReplace_StringStringArrayStringArrayBoolean() {
//JAVADOC TESTS START
assertNull(StringUtils.replaceEachRepeatedly(null, new String[]{"a"}, new String[]{"b"}));
assertEquals(StringUtils.replaceEachRepeatedly("", new String[]{"a"}, new String[]{"b"}),"");
assertEquals(StringUtils.replaceEachRepeatedly("aba", null, null),"aba");
assertEquals(StringUtils.replaceEachRepeatedly("aba", new String[0], null),"aba");
assertEquals(StringUtils.replaceEachRepeatedly("aba", null, new String[0]),"aba");
assertEquals(StringUtils.replaceEachRepeatedly("aba", new String[0], null),"aba");
assertEquals(StringUtils.replaceEachRepeatedly("aba", new String[]{"a"}, new String[]{""}),"b");
assertEquals(StringUtils.replaceEachRepeatedly("aba", new String[]{null}, new String[]{"a"}),"aba");
assertEquals(StringUtils.replaceEachRepeatedly("abcde", new String[]{"ab", "d"}, new String[]{"w", "t"}),"wcte");
assertEquals(StringUtils.replaceEachRepeatedly("abcde", new String[]{"ab", "d"}, new String[]{"d", "t"}),"tcte");
try {
StringUtils.replaceEachRepeatedly("abcde", new String[]{"ab", "d"}, new String[]{"d", "ab"});
fail("Should be a circular reference");
} catch (IllegalStateException e) {}
//JAVADOC TESTS END
}
public void testReplaceChars_StringCharChar() {
assertEquals(null, StringUtils.replaceChars(null, 'b', 'z'));
assertEquals("", StringUtils.replaceChars("", 'b', 'z'));
assertEquals("azcza", StringUtils.replaceChars("abcba", 'b', 'z'));
assertEquals("abcba", StringUtils.replaceChars("abcba", 'x', 'z'));
}
public void testReplaceChars_StringStringString() {
assertEquals(null, StringUtils.replaceChars(null, null, null));
assertEquals(null, StringUtils.replaceChars(null, "", null));
assertEquals(null, StringUtils.replaceChars(null, "a", null));
assertEquals(null, StringUtils.replaceChars(null, null, ""));
assertEquals(null, StringUtils.replaceChars(null, null, "x"));
assertEquals("", StringUtils.replaceChars("", null, null));
assertEquals("", StringUtils.replaceChars("", "", null));
assertEquals("", StringUtils.replaceChars("", "a", null));
assertEquals("", StringUtils.replaceChars("", null, ""));
assertEquals("", StringUtils.replaceChars("", null, "x"));
assertEquals("abc", StringUtils.replaceChars("abc", null, null));
assertEquals("abc", StringUtils.replaceChars("abc", null, ""));
assertEquals("abc", StringUtils.replaceChars("abc", null, "x"));
assertEquals("abc", StringUtils.replaceChars("abc", "", null));
assertEquals("abc", StringUtils.replaceChars("abc", "", ""));
assertEquals("abc", StringUtils.replaceChars("abc", "", "x"));
assertEquals("ac", StringUtils.replaceChars("abc", "b", null));
assertEquals("ac", StringUtils.replaceChars("abc", "b", ""));
assertEquals("axc", StringUtils.replaceChars("abc", "b", "x"));
assertEquals("ayzya", StringUtils.replaceChars("abcba", "bc", "yz"));
assertEquals("ayya", StringUtils.replaceChars("abcba", "bc", "y"));
assertEquals("ayzya", StringUtils.replaceChars("abcba", "bc", "yzx"));
assertEquals("abcba", StringUtils.replaceChars("abcba", "z", "w"));
assertSame("abcba", StringUtils.replaceChars("abcba", "z", "w"));
// Javadoc examples:
assertEquals("jelly", StringUtils.replaceChars("hello", "ho", "jy"));
assertEquals("ayzya", StringUtils.replaceChars("abcba", "bc", "yz"));
assertEquals("ayya", StringUtils.replaceChars("abcba", "bc", "y"));
assertEquals("ayzya", StringUtils.replaceChars("abcba", "bc", "yzx"));
// From http://issues.apache.org/bugzilla/show_bug.cgi?id=25454
assertEquals("bcc", StringUtils.replaceChars("abc", "ab", "bc"));
assertEquals("q651.506bera", StringUtils.replaceChars("d216.102oren",
"abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ123456789",
"nopqrstuvwxyzabcdefghijklmNOPQRSTUVWXYZABCDEFGHIJKLM567891234"));
}
public void testOverlay_StringStringIntInt() {
assertEquals(null, StringUtils.overlay(null, null, 2, 4));
assertEquals(null, StringUtils.overlay(null, null, -2, -4));
assertEquals("", StringUtils.overlay("", null, 0, 0));
assertEquals("", StringUtils.overlay("", "", 0, 0));
assertEquals("zzzz", StringUtils.overlay("", "zzzz", 0, 0));
assertEquals("zzzz", StringUtils.overlay("", "zzzz", 2, 4));
assertEquals("zzzz", StringUtils.overlay("", "zzzz", -2, -4));
assertEquals("abef", StringUtils.overlay("abcdef", null, 2, 4));
assertEquals("abef", StringUtils.overlay("abcdef", null, 4, 2));
assertEquals("abef", StringUtils.overlay("abcdef", "", 2, 4));
assertEquals("abef", StringUtils.overlay("abcdef", "", 4, 2));
assertEquals("abzzzzef", StringUtils.overlay("abcdef", "zzzz", 2, 4));
assertEquals("abzzzzef", StringUtils.overlay("abcdef", "zzzz", 4, 2));
assertEquals("zzzzef", StringUtils.overlay("abcdef", "zzzz", -1, 4));
assertEquals("zzzzef", StringUtils.overlay("abcdef", "zzzz", 4, -1));
assertEquals("zzzzabcdef", StringUtils.overlay("abcdef", "zzzz", -2, -1));
assertEquals("zzzzabcdef", StringUtils.overlay("abcdef", "zzzz", -1, -2));
assertEquals("abcdzzzz", StringUtils.overlay("abcdef", "zzzz", 4, 10));
assertEquals("abcdzzzz", StringUtils.overlay("abcdef", "zzzz", 10, 4));
assertEquals("abcdefzzzz", StringUtils.overlay("abcdef", "zzzz", 8, 10));
assertEquals("abcdefzzzz", StringUtils.overlay("abcdef", "zzzz", 10, 8));
}
public void testRepeat_StringInt() {
assertEquals(null, StringUtils.repeat(null, 2));
assertEquals("", StringUtils.repeat("ab", 0));
assertEquals("", StringUtils.repeat("", 3));
assertEquals("aaa", StringUtils.repeat("a", 3));
assertEquals("ababab", StringUtils.repeat("ab", 3));
assertEquals("abcabcabc", StringUtils.repeat("abc", 3));
String str = StringUtils.repeat("a", 10000); // bigger than pad limit
assertEquals(10000, str.length());
assertEquals(true, StringUtils.containsOnly(str, new char[] {'a'}));
}
public void testRepeat_StringStringInt() {
assertEquals(null, StringUtils.repeat(null, null, 2));
assertEquals(null, StringUtils.repeat(null, "x", 2));
assertEquals("", StringUtils.repeat("", null, 2));
assertEquals("", StringUtils.repeat("ab", "", 0));
assertEquals("", StringUtils.repeat("", "", 2));
assertEquals("xx", StringUtils.repeat("", "x", 3));
assertEquals("?, ?, ?", StringUtils.repeat("?", ", ", 3));
}
public void testChop() {
String[][] chopCases = {
{ FOO_UNCAP + "\r\n", FOO_UNCAP } ,
{ FOO_UNCAP + "\n" , FOO_UNCAP } ,
{ FOO_UNCAP + "\r", FOO_UNCAP },
{ FOO_UNCAP + " \r", FOO_UNCAP + " " },
{ "foo", "fo"},
{ "foo\nfoo", "foo\nfo" },
{ "\n", "" },
{ "\r", "" },
{ "\r\n", "" },
{ null, null },
{ "", "" },
{ "a", "" },
};
for (int i = 0; i < chopCases.length; i++) {
String original = chopCases[i][0];
String expectedResult = chopCases[i][1];
assertEquals("chop(String) failed",
expectedResult, StringUtils.chop(original));
}
}
public void testChomp() {
String[][] chompCases = {
{ FOO_UNCAP + "\r\n", FOO_UNCAP },
{ FOO_UNCAP + "\n" , FOO_UNCAP },
{ FOO_UNCAP + "\r", FOO_UNCAP },
{ FOO_UNCAP + " \r", FOO_UNCAP + " " },
{ FOO_UNCAP, FOO_UNCAP },
{ FOO_UNCAP + "\n\n", FOO_UNCAP + "\n"},
{ FOO_UNCAP + "\r\n\r\n", FOO_UNCAP + "\r\n" },
{ "foo\nfoo", "foo\nfoo" },
{ "foo\n\rfoo", "foo\n\rfoo" },
{ "\n", "" },
{ "\r", "" },
{ "a", "a" },
{ "\r\n", "" },
{ "", "" },
{ null, null },
{ FOO_UNCAP + "\n\r", FOO_UNCAP + "\n"}
};
for (int i = 0; i < chompCases.length; i++) {
String original = chompCases[i][0];
String expectedResult = chompCases[i][1];
assertEquals("chomp(String) failed",
expectedResult, StringUtils.chomp(original));
}
assertEquals("chomp(String, String) failed",
"foo", StringUtils.chomp("foobar", "bar"));
assertEquals("chomp(String, String) failed",
"foobar", StringUtils.chomp("foobar", "baz"));
assertEquals("chomp(String, String) failed",
"foo", StringUtils.chomp("foo", "foooo"));
assertEquals("chomp(String, String) failed",
"foobar", StringUtils.chomp("foobar", ""));
assertEquals("chomp(String, String) failed",
"foobar", StringUtils.chomp("foobar", null));
assertEquals("chomp(String, String) failed",
"", StringUtils.chomp("", "foo"));
assertEquals("chomp(String, String) failed",
"", StringUtils.chomp("", null));
assertEquals("chomp(String, String) failed",
"", StringUtils.chomp("", ""));
assertEquals("chomp(String, String) failed",
null, StringUtils.chomp(null, "foo"));
assertEquals("chomp(String, String) failed",
null, StringUtils.chomp(null, null));
assertEquals("chomp(String, String) failed",
null, StringUtils.chomp(null, ""));
assertEquals("chomp(String, String) failed",
"", StringUtils.chomp("foo", "foo"));
assertEquals("chomp(String, String) failed",
" ", StringUtils.chomp(" foo", "foo"));
assertEquals("chomp(String, String) failed",
"foo ", StringUtils.chomp("foo ", "foo"));
}
//-----------------------------------------------------------------------
public void testRightPad_StringInt() {
assertEquals(null, StringUtils.rightPad(null, 5));
assertEquals(" ", StringUtils.rightPad("", 5));
assertEquals("abc ", StringUtils.rightPad("abc", 5));
assertEquals("abc", StringUtils.rightPad("abc", 2));
assertEquals("abc", StringUtils.rightPad("abc", -1));
}
public void testRightPad_StringIntChar() {
assertEquals(null, StringUtils.rightPad(null, 5, ' '));
assertEquals(" ", StringUtils.rightPad("", 5, ' '));
assertEquals("abc ", StringUtils.rightPad("abc", 5, ' '));
assertEquals("abc", StringUtils.rightPad("abc", 2, ' '));
assertEquals("abc", StringUtils.rightPad("abc", -1, ' '));
assertEquals("abcxx", StringUtils.rightPad("abc", 5, 'x'));
String str = StringUtils.rightPad("aaa", 10000, 'a'); // bigger than pad length
assertEquals(10000, str.length());
assertEquals(true, StringUtils.containsOnly(str, new char[] {'a'}));
}
public void testRightPad_StringIntString() {
assertEquals(null, StringUtils.rightPad(null, 5, "-+"));
assertEquals(" ", StringUtils.rightPad("", 5, " "));
assertEquals(null, StringUtils.rightPad(null, 8, null));
assertEquals("abc-+-+", StringUtils.rightPad("abc", 7, "-+"));
assertEquals("abc-+~", StringUtils.rightPad("abc", 6, "-+~"));
assertEquals("abc-+", StringUtils.rightPad("abc", 5, "-+~"));
assertEquals("abc", StringUtils.rightPad("abc", 2, " "));
assertEquals("abc", StringUtils.rightPad("abc", -1, " "));
assertEquals("abc ", StringUtils.rightPad("abc", 5, null));
assertEquals("abc ", StringUtils.rightPad("abc", 5, ""));
}
//-----------------------------------------------------------------------
public void testLeftPad_StringInt() {
assertEquals(null, StringUtils.leftPad(null, 5));
assertEquals(" ", StringUtils.leftPad("", 5));
assertEquals(" abc", StringUtils.leftPad("abc", 5));
assertEquals("abc", StringUtils.leftPad("abc", 2));
}
public void testLeftPad_StringIntChar() {
assertEquals(null, StringUtils.leftPad(null, 5, ' '));
assertEquals(" ", StringUtils.leftPad("", 5, ' '));
assertEquals(" abc", StringUtils.leftPad("abc", 5, ' '));
assertEquals("xxabc", StringUtils.leftPad("abc", 5, 'x'));
assertEquals("\uffff\uffffabc", StringUtils.leftPad("abc", 5, '\uffff'));
assertEquals("abc", StringUtils.leftPad("abc", 2, ' '));
String str = StringUtils.leftPad("aaa", 10000, 'a'); // bigger than pad length
assertEquals(10000, str.length());
assertEquals(true, StringUtils.containsOnly(str, new char[] {'a'}));
}
public void testLeftPad_StringIntString() {
assertEquals(null, StringUtils.leftPad(null, 5, "-+"));
assertEquals(null, StringUtils.leftPad(null, 5, null));
assertEquals(" ", StringUtils.leftPad("", 5, " "));
assertEquals("-+-+abc", StringUtils.leftPad("abc", 7, "-+"));
assertEquals("-+~abc", StringUtils.leftPad("abc", 6, "-+~"));
assertEquals("-+abc", StringUtils.leftPad("abc", 5, "-+~"));
assertEquals("abc", StringUtils.leftPad("abc", 2, " "));
assertEquals("abc", StringUtils.leftPad("abc", -1, " "));
assertEquals(" abc", StringUtils.leftPad("abc", 5, null));
assertEquals(" abc", StringUtils.leftPad("abc", 5, ""));
}
public void testLength() {
assertEquals(0, StringUtils.length(null));
assertEquals(0, StringUtils.length(""));
assertEquals(0, StringUtils.length(StringUtils.EMPTY));
assertEquals(1, StringUtils.length("A"));
assertEquals(1, StringUtils.length(" "));
assertEquals(8, StringUtils.length("ABCDEFGH"));
}
//-----------------------------------------------------------------------
public void testCenter_StringInt() {
assertEquals(null, StringUtils.center(null, -1));
assertEquals(null, StringUtils.center(null, 4));
assertEquals(" ", StringUtils.center("", 4));
assertEquals("ab", StringUtils.center("ab", 0));
assertEquals("ab", StringUtils.center("ab", -1));
assertEquals("ab", StringUtils.center("ab", 1));
assertEquals(" ", StringUtils.center("", 4));
assertEquals(" ab ", StringUtils.center("ab", 4));
assertEquals("abcd", StringUtils.center("abcd", 2));
assertEquals(" a ", StringUtils.center("a", 4));
assertEquals(" a ", StringUtils.center("a", 5));
}
public void testCenter_StringIntChar() {
assertEquals(null, StringUtils.center(null, -1, ' '));
assertEquals(null, StringUtils.center(null, 4, ' '));
assertEquals(" ", StringUtils.center("", 4, ' '));
assertEquals("ab", StringUtils.center("ab", 0, ' '));
assertEquals("ab", StringUtils.center("ab", -1, ' '));
assertEquals("ab", StringUtils.center("ab", 1, ' '));
assertEquals(" ", StringUtils.center("", 4, ' '));
assertEquals(" ab ", StringUtils.center("ab", 4, ' '));
assertEquals("abcd", StringUtils.center("abcd", 2, ' '));
assertEquals(" a ", StringUtils.center("a", 4, ' '));
assertEquals(" a ", StringUtils.center("a", 5, ' '));
assertEquals("xxaxx", StringUtils.center("a", 5, 'x'));
}
public void testCenter_StringIntString() {
assertEquals(null, StringUtils.center(null, 4, null));
assertEquals(null, StringUtils.center(null, -1, " "));
assertEquals(null, StringUtils.center(null, 4, " "));
assertEquals(" ", StringUtils.center("", 4, " "));
assertEquals("ab", StringUtils.center("ab", 0, " "));
assertEquals("ab", StringUtils.center("ab", -1, " "));
assertEquals("ab", StringUtils.center("ab", 1, " "));
assertEquals(" ", StringUtils.center("", 4, " "));
assertEquals(" ab ", StringUtils.center("ab", 4, " "));
assertEquals("abcd", StringUtils.center("abcd", 2, " "));
assertEquals(" a ", StringUtils.center("a", 4, " "));
assertEquals("yayz", StringUtils.center("a", 4, "yz"));
assertEquals("yzyayzy", StringUtils.center("a", 7, "yz"));
assertEquals(" abc ", StringUtils.center("abc", 7, null));
assertEquals(" abc ", StringUtils.center("abc", 7, ""));
}
//-----------------------------------------------------------------------
public void testReverse_String() {
assertEquals(null, StringUtils.reverse(null) );
assertEquals("", StringUtils.reverse("") );
assertEquals("sdrawkcab", StringUtils.reverse("backwards") );
}
public void testReverseDelimited_StringChar() {
assertEquals(null, StringUtils.reverseDelimited(null, '.') );
assertEquals("", StringUtils.reverseDelimited("", '.') );
assertEquals("c.b.a", StringUtils.reverseDelimited("a.b.c", '.') );
assertEquals("a b c", StringUtils.reverseDelimited("a b c", '.') );
assertEquals("", StringUtils.reverseDelimited("", '.') );
}
//-----------------------------------------------------------------------
public void testDefault_String() {
assertEquals("", StringUtils.defaultString(null));
assertEquals("", StringUtils.defaultString(""));
assertEquals("abc", StringUtils.defaultString("abc"));
}
public void testDefault_StringString() {
assertEquals("NULL", StringUtils.defaultString(null, "NULL"));
assertEquals("", StringUtils.defaultString("", "NULL"));
assertEquals("abc", StringUtils.defaultString("abc", "NULL"));
}
public void testDefaultIfEmpty_StringString() {
assertEquals("NULL", StringUtils.defaultIfEmpty(null, "NULL"));
assertEquals("NULL", StringUtils.defaultIfEmpty("", "NULL"));
assertEquals("abc", StringUtils.defaultIfEmpty("abc", "NULL"));
assertNull(StringUtils.defaultIfEmpty("", null));
}
//-----------------------------------------------------------------------
public void testAbbreviate_StringInt() {
assertEquals(null, StringUtils.abbreviate(null, 10));
assertEquals("", StringUtils.abbreviate("", 10));
assertEquals("short", StringUtils.abbreviate("short", 10));
assertEquals("Now is ...", StringUtils.abbreviate("Now is the time for all good men to come to the aid of their party.", 10));
String raspberry = "raspberry peach";
assertEquals("raspberry p...", StringUtils.abbreviate(raspberry, 14));
assertEquals("raspberry peach", StringUtils.abbreviate("raspberry peach", 15));
assertEquals("raspberry peach", StringUtils.abbreviate("raspberry peach", 16));
assertEquals("abc...", StringUtils.abbreviate("abcdefg", 6));
assertEquals("abcdefg", StringUtils.abbreviate("abcdefg", 7));
assertEquals("abcdefg", StringUtils.abbreviate("abcdefg", 8));
assertEquals("a...", StringUtils.abbreviate("abcdefg", 4));
assertEquals("", StringUtils.abbreviate("", 4));
try {
@SuppressWarnings("unused")
String res = StringUtils.abbreviate("abc", 3);
fail("StringUtils.abbreviate expecting IllegalArgumentException");
} catch (IllegalArgumentException ex) {
// empty
}
}
public void testAbbreviate_StringIntInt() {
assertEquals(null, StringUtils.abbreviate(null, 10, 12));
assertEquals("", StringUtils.abbreviate("", 0, 10));
assertEquals("", StringUtils.abbreviate("", 2, 10));
try {
@SuppressWarnings("unused")
String res = StringUtils.abbreviate("abcdefghij", 0, 3);
fail("StringUtils.abbreviate expecting IllegalArgumentException");
} catch (IllegalArgumentException ex) {
// empty
}
try {
@SuppressWarnings("unused")
String res = StringUtils.abbreviate("abcdefghij", 5, 6);
fail("StringUtils.abbreviate expecting IllegalArgumentException");
} catch (IllegalArgumentException ex) {
// empty
}
String raspberry = "raspberry peach";
assertEquals("raspberry peach", StringUtils.abbreviate(raspberry, 11, 15));
assertEquals(null, StringUtils.abbreviate(null, 7, 14));
assertAbbreviateWithOffset("abcdefg...", -1, 10);
assertAbbreviateWithOffset("abcdefg...", 0, 10);
assertAbbreviateWithOffset("abcdefg...", 1, 10);
assertAbbreviateWithOffset("abcdefg...", 2, 10);
assertAbbreviateWithOffset("abcdefg...", 3, 10);
assertAbbreviateWithOffset("abcdefg...", 4, 10);
assertAbbreviateWithOffset("...fghi...", 5, 10);
assertAbbreviateWithOffset("...ghij...", 6, 10);
assertAbbreviateWithOffset("...hijk...", 7, 10);
assertAbbreviateWithOffset("...ijklmno", 8, 10);
assertAbbreviateWithOffset("...ijklmno", 9, 10);
assertAbbreviateWithOffset("...ijklmno", 10, 10);
assertAbbreviateWithOffset("...ijklmno", 10, 10);
assertAbbreviateWithOffset("...ijklmno", 11, 10);
assertAbbreviateWithOffset("...ijklmno", 12, 10);
assertAbbreviateWithOffset("...ijklmno", 13, 10);
assertAbbreviateWithOffset("...ijklmno", 14, 10);
assertAbbreviateWithOffset("...ijklmno", 15, 10);
assertAbbreviateWithOffset("...ijklmno", 16, 10);
assertAbbreviateWithOffset("...ijklmno", Integer.MAX_VALUE, 10);
}
private void assertAbbreviateWithOffset(String expected, int offset, int maxWidth) {
String abcdefghijklmno = "abcdefghijklmno";
String message = "abbreviate(String,int,int) failed";
String actual = StringUtils.abbreviate(abcdefghijklmno, offset, maxWidth);
if (offset >= 0 && offset < abcdefghijklmno.length()) {
assertTrue(message + " -- should contain offset character",
actual.indexOf((char)('a'+offset)) != -1);
}
assertTrue(message + " -- should not be greater than maxWidth",
actual.length() <= maxWidth);
assertEquals(message, expected, actual);
}
//-----------------------------------------------------------------------
public void testDifference_StringString() {
assertEquals(null, StringUtils.difference(null, null));
assertEquals("", StringUtils.difference("", ""));
assertEquals("abc", StringUtils.difference("", "abc"));
assertEquals("", StringUtils.difference("abc", ""));
assertEquals("i am a robot", StringUtils.difference(null, "i am a robot"));
assertEquals("i am a machine", StringUtils.difference("i am a machine", null));
assertEquals("robot", StringUtils.difference("i am a machine", "i am a robot"));
assertEquals("", StringUtils.difference("abc", "abc"));
assertEquals("you are a robot", StringUtils.difference("i am a robot", "you are a robot"));
}
public void testDifferenceAt_StringString() {
assertEquals(-1, StringUtils.indexOfDifference(null, null));
assertEquals(0, StringUtils.indexOfDifference(null, "i am a robot"));
assertEquals(-1, StringUtils.indexOfDifference("", ""));
assertEquals(0, StringUtils.indexOfDifference("", "abc"));
assertEquals(0, StringUtils.indexOfDifference("abc", ""));
assertEquals(0, StringUtils.indexOfDifference("i am a machine", null));
assertEquals(7, StringUtils.indexOfDifference("i am a machine", "i am a robot"));
assertEquals(-1, StringUtils.indexOfDifference("foo", "foo"));
assertEquals(0, StringUtils.indexOfDifference("i am a robot", "you are a robot"));
//System.out.println("indexOfDiff: " + StringUtils.indexOfDifference("i am a robot", "not machine"));
}
//-----------------------------------------------------------------------
public void testGetLevenshteinDistance_StringString() {
assertEquals(0, StringUtils.getLevenshteinDistance("", "") );
assertEquals(1, StringUtils.getLevenshteinDistance("", "a") );
assertEquals(7, StringUtils.getLevenshteinDistance("aaapppp", "") );
assertEquals(1, StringUtils.getLevenshteinDistance("frog", "fog") );
assertEquals(3, StringUtils.getLevenshteinDistance("fly", "ant") );
assertEquals(7, StringUtils.getLevenshteinDistance("elephant", "hippo") );
assertEquals(7, StringUtils.getLevenshteinDistance("hippo", "elephant") );
assertEquals(8, StringUtils.getLevenshteinDistance("hippo", "zzzzzzzz") );
assertEquals(8, StringUtils.getLevenshteinDistance("zzzzzzzz", "hippo") );
assertEquals(1, StringUtils.getLevenshteinDistance("hello", "hallo") );
try {
@SuppressWarnings("unused")
int d = StringUtils.getLevenshteinDistance("a", null);
fail("expecting IllegalArgumentException");
} catch (IllegalArgumentException ex) {
// empty
}
try {
@SuppressWarnings("unused")
int d = StringUtils.getLevenshteinDistance(null, "a");
fail("expecting IllegalArgumentException");
} catch (IllegalArgumentException ex) {
// empty
}
}
/**
* A sanity check for {@link StringUtils#EMPTY}.
*/
public void testEMPTY() {
assertNotNull(StringUtils.EMPTY);
assertEquals("", StringUtils.EMPTY);
assertEquals(0, StringUtils.EMPTY.length());
}
/**
* Test for {@link StringUtils#isAllLowerCase(String)}.
*/
public void testIsAllLowerCase() {
assertFalse(StringUtils.isAllLowerCase(null));
assertFalse(StringUtils.isAllLowerCase(StringUtils.EMPTY));
assertTrue(StringUtils.isAllLowerCase("abc"));
assertFalse(StringUtils.isAllLowerCase("abc "));
assertFalse(StringUtils.isAllLowerCase("abC"));
}
/**
* Test for {@link StringUtils#isAllUpperCase(String)}.
*/
public void testIsAllUpperCase() {
assertFalse(StringUtils.isAllUpperCase(null));
assertFalse(StringUtils.isAllUpperCase(StringUtils.EMPTY));
assertTrue(StringUtils.isAllUpperCase("ABC"));
assertFalse(StringUtils.isAllUpperCase("ABC "));
assertFalse(StringUtils.isAllUpperCase("aBC"));
}
public void testRemoveStart() {
// StringUtils.removeStart("", *) = ""
assertNull(StringUtils.removeStart(null, null));
assertNull(StringUtils.removeStart(null, ""));
assertNull(StringUtils.removeStart(null, "a"));
// StringUtils.removeStart(*, null) = *
assertEquals(StringUtils.removeStart("", null), "");
assertEquals(StringUtils.removeStart("", ""), "");
assertEquals(StringUtils.removeStart("", "a"), "");
// All others:
assertEquals(StringUtils.removeStart("www.domain.com", "www."), "domain.com");
assertEquals(StringUtils.removeStart("domain.com", "www."), "domain.com");
assertEquals(StringUtils.removeStart("domain.com", ""), "domain.com");
assertEquals(StringUtils.removeStart("domain.com", null), "domain.com");
}
public void testRemoveStartIgnoreCase() {
// StringUtils.removeStart("", *) = ""
assertNull("removeStartIgnoreCase(null, null)", StringUtils.removeStartIgnoreCase(null, null));
assertNull("removeStartIgnoreCase(null, \"\")", StringUtils.removeStartIgnoreCase(null, ""));
assertNull("removeStartIgnoreCase(null, \"a\")", StringUtils.removeStartIgnoreCase(null, "a"));
// StringUtils.removeStart(*, null) = *
assertEquals("removeStartIgnoreCase(\"\", null)", StringUtils.removeStartIgnoreCase("", null), "");
assertEquals("removeStartIgnoreCase(\"\", \"\")", StringUtils.removeStartIgnoreCase("", ""), "");
assertEquals("removeStartIgnoreCase(\"\", \"a\")", StringUtils.removeStartIgnoreCase("", "a"), "");
// All others:
assertEquals("removeStartIgnoreCase(\"www.domain.com\", \"www.\")", StringUtils.removeStartIgnoreCase("www.domain.com", "www."), "domain.com");
assertEquals("removeStartIgnoreCase(\"domain.com\", \"www.\")", StringUtils.removeStartIgnoreCase("domain.com", "www."), "domain.com");
assertEquals("removeStartIgnoreCase(\"domain.com\", \"\")", StringUtils.removeStartIgnoreCase("domain.com", ""), "domain.com");
assertEquals("removeStartIgnoreCase(\"domain.com\", null)", StringUtils.removeStartIgnoreCase("domain.com", null), "domain.com");
// Case insensitive:
assertEquals("removeStartIgnoreCase(\"www.domain.com\", \"WWW.\")", StringUtils.removeStartIgnoreCase("www.domain.com", "WWW."), "domain.com");
}
public void testRemoveEnd() {
// StringUtils.removeEnd("", *) = ""
assertNull(StringUtils.removeEnd(null, null));
assertNull(StringUtils.removeEnd(null, ""));
assertNull(StringUtils.removeEnd(null, "a"));
// StringUtils.removeEnd(*, null) = *
assertEquals(StringUtils.removeEnd("", null), "");
assertEquals(StringUtils.removeEnd("", ""), "");
assertEquals(StringUtils.removeEnd("", "a"), "");
// All others:
assertEquals(StringUtils.removeEnd("www.domain.com.", ".com"), "www.domain.com.");
assertEquals(StringUtils.removeEnd("www.domain.com", ".com"), "www.domain");
assertEquals(StringUtils.removeEnd("www.domain", ".com"), "www.domain");
assertEquals(StringUtils.removeEnd("domain.com", ""), "domain.com");
assertEquals(StringUtils.removeEnd("domain.com", null), "domain.com");
}
public void testRemoveEndIgnoreCase() {
// StringUtils.removeEndIgnoreCase("", *) = ""
assertNull("removeEndIgnoreCase(null, null)", StringUtils.removeEndIgnoreCase(null, null));
assertNull("removeEndIgnoreCase(null, \"\")", StringUtils.removeEndIgnoreCase(null, ""));
assertNull("removeEndIgnoreCase(null, \"a\")", StringUtils.removeEndIgnoreCase(null, "a"));
// StringUtils.removeEnd(*, null) = *
assertEquals("removeEndIgnoreCase(\"\", null)", StringUtils.removeEndIgnoreCase("", null), "");
assertEquals("removeEndIgnoreCase(\"\", \"\")", StringUtils.removeEndIgnoreCase("", ""), "");
assertEquals("removeEndIgnoreCase(\"\", \"a\")", StringUtils.removeEndIgnoreCase("", "a"), "");
// All others:
assertEquals("removeEndIgnoreCase(\"www.domain.com.\", \".com\")", StringUtils.removeEndIgnoreCase("www.domain.com.", ".com"), "www.domain.com.");
assertEquals("removeEndIgnoreCase(\"www.domain.com\", \".com\")", StringUtils.removeEndIgnoreCase("www.domain.com", ".com"), "www.domain");
assertEquals("removeEndIgnoreCase(\"www.domain\", \".com\")", StringUtils.removeEndIgnoreCase("www.domain", ".com"), "www.domain");
assertEquals("removeEndIgnoreCase(\"domain.com\", \"\")", StringUtils.removeEndIgnoreCase("domain.com", ""), "domain.com");
assertEquals("removeEndIgnoreCase(\"domain.com\", null)", StringUtils.removeEndIgnoreCase("domain.com", null), "domain.com");
// Case insensitive:
assertEquals("removeEndIgnoreCase(\"www.domain.com\", \".com\")", StringUtils.removeEndIgnoreCase("www.domain.com", ".COM"), "www.domain");
}
public void testRemove_String() {
// StringUtils.remove(null, *) = null
assertEquals(null, StringUtils.remove(null, null));
assertEquals(null, StringUtils.remove(null, ""));
assertEquals(null, StringUtils.remove(null, "a"));
// StringUtils.remove("", *) = ""
assertEquals("", StringUtils.remove("", null));
assertEquals("", StringUtils.remove("", ""));
assertEquals("", StringUtils.remove("", "a"));
// StringUtils.remove(*, null) = *
assertEquals(null, StringUtils.remove(null, null));
assertEquals("", StringUtils.remove("", null));
assertEquals("a", StringUtils.remove("a", null));
// StringUtils.remove(*, "") = *
assertEquals(null, StringUtils.remove(null, ""));
assertEquals("", StringUtils.remove("", ""));
assertEquals("a", StringUtils.remove("a", ""));
// StringUtils.remove("queued", "ue") = "qd"
assertEquals("qd", StringUtils.remove("queued", "ue"));
// StringUtils.remove("queued", "zz") = "queued"
assertEquals("queued", StringUtils.remove("queued", "zz"));
}
public void testRemove_char() {
// StringUtils.remove(null, *) = null
assertEquals(null, StringUtils.remove(null, 'a'));
assertEquals(null, StringUtils.remove(null, 'a'));
assertEquals(null, StringUtils.remove(null, 'a'));
// StringUtils.remove("", *) = ""
assertEquals("", StringUtils.remove("", 'a'));
assertEquals("", StringUtils.remove("", 'a'));
assertEquals("", StringUtils.remove("", 'a'));
// StringUtils.remove("queued", 'u') = "qeed"
assertEquals("qeed", StringUtils.remove("queued", 'u'));
// StringUtils.remove("queued", 'z') = "queued"
assertEquals("queued", StringUtils.remove("queued", 'z'));
}
public void testDifferenceAt_StringArray(){
assertEquals(-1, StringUtils.indexOfDifference(null));
assertEquals(-1, StringUtils.indexOfDifference(new String[] {}));
assertEquals(-1, StringUtils.indexOfDifference(new String[] {"abc"}));
assertEquals(-1, StringUtils.indexOfDifference(new String[] {null, null}));
assertEquals(-1, StringUtils.indexOfDifference(new String[] {"", ""}));
assertEquals(0, StringUtils.indexOfDifference(new String[] {"", null}));
assertEquals(0, StringUtils.indexOfDifference(new String[] {"abc", null, null}));
assertEquals(0, StringUtils.indexOfDifference(new String[] {null, null, "abc"}));
assertEquals(0, StringUtils.indexOfDifference(new String[] {"", "abc"}));
assertEquals(0, StringUtils.indexOfDifference(new String[] {"abc", ""}));
assertEquals(-1, StringUtils.indexOfDifference(new String[] {"abc", "abc"}));
assertEquals(1, StringUtils.indexOfDifference(new String[] {"abc", "a"}));
assertEquals(2, StringUtils.indexOfDifference(new String[] {"ab", "abxyz"}));
assertEquals(2, StringUtils.indexOfDifference(new String[] {"abcde", "abxyz"}));
assertEquals(0, StringUtils.indexOfDifference(new String[] {"abcde", "xyz"}));
assertEquals(0, StringUtils.indexOfDifference(new String[] {"xyz", "abcde"}));
assertEquals(7, StringUtils.indexOfDifference(new String[] {"i am a machine", "i am a robot"}));
}
public void testGetCommonPrefix_StringArray(){
assertEquals("", StringUtils.getCommonPrefix(null));
assertEquals("", StringUtils.getCommonPrefix(new String[] {}));
assertEquals("abc", StringUtils.getCommonPrefix(new String[] {"abc"}));
assertEquals("", StringUtils.getCommonPrefix(new String[] {null, null}));
assertEquals("", StringUtils.getCommonPrefix(new String[] {"", ""}));
assertEquals("", StringUtils.getCommonPrefix(new String[] {"", null}));
assertEquals("", StringUtils.getCommonPrefix(new String[] {"abc", null, null}));
assertEquals("", StringUtils.getCommonPrefix(new String[] {null, null, "abc"}));
assertEquals("", StringUtils.getCommonPrefix(new String[] {"", "abc"}));
assertEquals("", StringUtils.getCommonPrefix(new String[] {"abc", ""}));
assertEquals("abc", StringUtils.getCommonPrefix(new String[] {"abc", "abc"}));
assertEquals("a", StringUtils.getCommonPrefix(new String[] {"abc", "a"}));
assertEquals("ab", StringUtils.getCommonPrefix(new String[] {"ab", "abxyz"}));
assertEquals("ab", StringUtils.getCommonPrefix(new String[] {"abcde", "abxyz"}));
assertEquals("", StringUtils.getCommonPrefix(new String[] {"abcde", "xyz"}));
assertEquals("", StringUtils.getCommonPrefix(new String[] {"xyz", "abcde"}));
assertEquals("i am a ", StringUtils.getCommonPrefix(new String[] {"i am a machine", "i am a robot"}));
}
public void testStartsWithAny() {
assertFalse(StringUtils.startsWithAny(null, null));
assertFalse(StringUtils.startsWithAny(null, new String[] {"abc"}));
assertFalse(StringUtils.startsWithAny("abcxyz", null));
assertFalse(StringUtils.startsWithAny("abcxyz", new String[] {}));
assertTrue(StringUtils.startsWithAny("abcxyz", new String[] {"abc"}));
assertTrue(StringUtils.startsWithAny("abcxyz", new String[] {null, "xyz", "abc"}));
assertFalse(StringUtils.startsWithAny("abcxyz", new String[] {null, "xyz", "abcd"}));
}
} | // You are a professional Java test case writer, please create a test case named `testReplace_StringStringArrayStringArray` for the issue `Lang-LANG-552`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Lang-LANG-552
//
// ## Issue-Title:
// StringUtils replaceEach - Bug or Missing Documentation
//
// ## Issue-Description:
//
// The following Test Case for replaceEach fails with a null pointer exception.
//
// I have expected that all StringUtils methods are "null-friendly"
//
// The use case is that i will stuff Values into the replacementList of which I do not want to check whether they are null.
//
// I admit the use case is not perfect, because it is unclear what happens on the replace.
//
// I outlined three expectations in the test case, of course only one should be met.
//
//
// If it is decided that none of them should be possible, I propose to update the documentation with what happens when null is passed as replacement string
//
//
//
//
// ```
// import static org.junit.Assert.assertEquals;
//
// import org.apache.commons.lang.StringUtils;
// import org.junit.Test;
//
//
// public class StringUtilsTest {
//
// @Test
// public void replaceEach(){
// String original = "Hello World!";
// String[] searchList = {"Hello", "World"};
// String[] replacementList = {"Greetings", null};
// String result = StringUtils.replaceEach(original, searchList, replacementList);
// assertEquals("Greetings !", result);
// //perhaps this is ok as well
// //assertEquals("Greetings World!", result);
// //or even
// //assertEquals("Greetings null!", result);
// }
//
//
// }
//
// ```
//
//
//
//
//
public void testReplace_StringStringArrayStringArray() {
| 1,039 | /**
* Test method for 'StringUtils.replaceEach(String, String[], String[])'
*/ | 39 | 1,008 | src/test/org/apache/commons/lang3/StringUtilsTest.java | src/test | ```markdown
## Issue-ID: Lang-LANG-552
## Issue-Title:
StringUtils replaceEach - Bug or Missing Documentation
## Issue-Description:
The following Test Case for replaceEach fails with a null pointer exception.
I have expected that all StringUtils methods are "null-friendly"
The use case is that i will stuff Values into the replacementList of which I do not want to check whether they are null.
I admit the use case is not perfect, because it is unclear what happens on the replace.
I outlined three expectations in the test case, of course only one should be met.
If it is decided that none of them should be possible, I propose to update the documentation with what happens when null is passed as replacement string
```
import static org.junit.Assert.assertEquals;
import org.apache.commons.lang.StringUtils;
import org.junit.Test;
public class StringUtilsTest {
@Test
public void replaceEach(){
String original = "Hello World!";
String[] searchList = {"Hello", "World"};
String[] replacementList = {"Greetings", null};
String result = StringUtils.replaceEach(original, searchList, replacementList);
assertEquals("Greetings !", result);
//perhaps this is ok as well
//assertEquals("Greetings World!", result);
//or even
//assertEquals("Greetings null!", result);
}
}
```
```
You are a professional Java test case writer, please create a test case named `testReplace_StringStringArrayStringArray` for the issue `Lang-LANG-552`, utilizing the provided issue report information and the following function signature.
```java
public void testReplace_StringStringArrayStringArray() {
```
| 1,008 | [
"org.apache.commons.lang3.StringUtils"
] | 907801b3dbef9179d55baf26d710ca872b717b5282f71bfa51ee9f933f6e3b04 | public void testReplace_StringStringArrayStringArray() | // You are a professional Java test case writer, please create a test case named `testReplace_StringStringArrayStringArray` for the issue `Lang-LANG-552`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Lang-LANG-552
//
// ## Issue-Title:
// StringUtils replaceEach - Bug or Missing Documentation
//
// ## Issue-Description:
//
// The following Test Case for replaceEach fails with a null pointer exception.
//
// I have expected that all StringUtils methods are "null-friendly"
//
// The use case is that i will stuff Values into the replacementList of which I do not want to check whether they are null.
//
// I admit the use case is not perfect, because it is unclear what happens on the replace.
//
// I outlined three expectations in the test case, of course only one should be met.
//
//
// If it is decided that none of them should be possible, I propose to update the documentation with what happens when null is passed as replacement string
//
//
//
//
// ```
// import static org.junit.Assert.assertEquals;
//
// import org.apache.commons.lang.StringUtils;
// import org.junit.Test;
//
//
// public class StringUtilsTest {
//
// @Test
// public void replaceEach(){
// String original = "Hello World!";
// String[] searchList = {"Hello", "World"};
// String[] replacementList = {"Greetings", null};
// String result = StringUtils.replaceEach(original, searchList, replacementList);
// assertEquals("Greetings !", result);
// //perhaps this is ok as well
// //assertEquals("Greetings World!", result);
// //or even
// //assertEquals("Greetings null!", result);
// }
//
//
// }
//
// ```
//
//
//
//
//
| Lang | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.lang3;
import java.lang.reflect.Constructor;
import java.lang.reflect.Modifier;
import java.util.Arrays;
import java.util.Collections;
import java.util.Iterator;
import java.util.Locale;
import junit.framework.Test;
import junit.framework.TestCase;
import junit.framework.TestSuite;
import junit.textui.TestRunner;
/**
* Unit tests {@link org.apache.commons.lang3.StringUtils}.
*
* @author Apache Software Foundation
* @author Daniel L. Rall
* @author <a href="mailto:[email protected]">Ringo De Smet</a>
* @author <a href="mailto:[email protected]>Fredrik Westermarck</a>
* @author Holger Krauth
* @author <a href="[email protected]">Henning P. Schmiedehausen</a>
* @author Phil Steitz
* @author Gary D. Gregory
* @author Scott Johnson
* @author Al Chou
* @version $Id$
*/
public class StringUtilsTest extends TestCase {
static final String WHITESPACE;
static final String NON_WHITESPACE;
static final String TRIMMABLE;
static final String NON_TRIMMABLE;
static {
String ws = "";
String nws = "";
String tr = "";
String ntr = "";
for (int i = 0; i < Character.MAX_VALUE; i++) {
if (Character.isWhitespace((char) i)) {
ws += String.valueOf((char) i);
if (i > 32) {
ntr += String.valueOf((char) i);
}
} else if (i < 40) {
nws += String.valueOf((char) i);
}
}
for (int i = 0; i <= 32; i++) {
tr += String.valueOf((char) i);
}
WHITESPACE = ws;
NON_WHITESPACE = nws;
TRIMMABLE = tr;
NON_TRIMMABLE = ntr;
}
private static final String[] ARRAY_LIST = { "foo", "bar", "baz" };
private static final String[] EMPTY_ARRAY_LIST = {};
private static final String[] NULL_ARRAY_LIST = {null};
private static final String[] MIXED_ARRAY_LIST = {null, "", "foo"};
private static final Object[] MIXED_TYPE_LIST = {new String("foo"), new Long(2)};
private static final String SEPARATOR = ",";
private static final char SEPARATOR_CHAR = ';';
private static final String TEXT_LIST = "foo,bar,baz";
private static final String TEXT_LIST_CHAR = "foo;bar;baz";
private static final String TEXT_LIST_NOSEP = "foobarbaz";
private static final String FOO_UNCAP = "foo";
private static final String FOO_CAP = "Foo";
private static final String SENTENCE_UNCAP = "foo bar baz";
private static final String SENTENCE_CAP = "Foo Bar Baz";
public StringUtilsTest(String name) {
super(name);
}
public static void main(String[] args) {
TestRunner.run(suite());
}
public static Test suite() {
TestSuite suite = new TestSuite(StringUtilsTest.class);
suite.setName("StringUtilsTest Tests");
return suite;
}
@Override
protected void setUp() throws Exception {
super.setUp();
}
@Override
protected void tearDown() throws Exception {
super.tearDown();
}
//-----------------------------------------------------------------------
public void testConstructor() {
assertNotNull(new StringUtils());
Constructor<?>[] cons = StringUtils.class.getDeclaredConstructors();
assertEquals(1, cons.length);
assertEquals(true, Modifier.isPublic(cons[0].getModifiers()));
assertEquals(true, Modifier.isPublic(StringUtils.class.getModifiers()));
assertEquals(false, Modifier.isFinal(StringUtils.class.getModifiers()));
}
//-----------------------------------------------------------------------
public void testCaseFunctions() {
assertEquals(null, StringUtils.upperCase(null));
assertEquals(null, StringUtils.upperCase(null, Locale.ENGLISH));
assertEquals(null, StringUtils.lowerCase(null));
assertEquals(null, StringUtils.lowerCase(null, Locale.ENGLISH));
assertEquals(null, StringUtils.capitalize(null));
assertEquals(null, StringUtils.uncapitalize(null));
assertEquals("capitalize(empty-string) failed",
"", StringUtils.capitalize("") );
assertEquals("capitalize(single-char-string) failed",
"X", StringUtils.capitalize("x") );
assertEquals("uncapitalize(String) failed",
FOO_UNCAP, StringUtils.uncapitalize(FOO_CAP) );
assertEquals("uncapitalize(empty-string) failed",
"", StringUtils.uncapitalize("") );
assertEquals("uncapitalize(single-char-string) failed",
"x", StringUtils.uncapitalize("X") );
// reflection type of tests: Sentences.
assertEquals("uncapitalize(capitalize(String)) failed",
SENTENCE_UNCAP, StringUtils.uncapitalize(StringUtils.capitalize(SENTENCE_UNCAP)) );
assertEquals("capitalize(uncapitalize(String)) failed",
SENTENCE_CAP, StringUtils.capitalize(StringUtils.uncapitalize(SENTENCE_CAP)) );
// reflection type of tests: One word.
assertEquals("uncapitalize(capitalize(String)) failed",
FOO_UNCAP, StringUtils.uncapitalize(StringUtils.capitalize(FOO_UNCAP)) );
assertEquals("capitalize(uncapitalize(String)) failed",
FOO_CAP, StringUtils.capitalize(StringUtils.uncapitalize(FOO_CAP)) );
assertEquals("upperCase(String) failed",
"FOO TEST THING", StringUtils.upperCase("fOo test THING") );
assertEquals("upperCase(empty-string) failed",
"", StringUtils.upperCase("") );
assertEquals("lowerCase(String) failed",
"foo test thing", StringUtils.lowerCase("fOo test THING") );
assertEquals("lowerCase(empty-string) failed",
"", StringUtils.lowerCase("") );
assertEquals("upperCase(String, Locale) failed",
"FOO TEST THING", StringUtils.upperCase("fOo test THING", Locale.ENGLISH) );
assertEquals("upperCase(empty-string, Locale) failed",
"", StringUtils.upperCase("", Locale.ENGLISH) );
assertEquals("lowerCase(String, Locale) failed",
"foo test thing", StringUtils.lowerCase("fOo test THING", Locale.ENGLISH) );
assertEquals("lowerCase(empty-string, Locale) failed",
"", StringUtils.lowerCase("", Locale.ENGLISH) );
}
public void testSwapCase_String() {
assertEquals(null, StringUtils.swapCase(null));
assertEquals("", StringUtils.swapCase(""));
assertEquals(" ", StringUtils.swapCase(" "));
assertEquals("i", WordUtils.swapCase("I") );
assertEquals("I", WordUtils.swapCase("i") );
assertEquals("I AM HERE 123", StringUtils.swapCase("i am here 123") );
assertEquals("i aM hERE 123", StringUtils.swapCase("I Am Here 123") );
assertEquals("I AM here 123", StringUtils.swapCase("i am HERE 123") );
assertEquals("i am here 123", StringUtils.swapCase("I AM HERE 123") );
String test = "This String contains a TitleCase character: \u01C8";
String expect = "tHIS sTRING CONTAINS A tITLEcASE CHARACTER: \u01C9";
assertEquals(expect, WordUtils.swapCase(test));
}
//-----------------------------------------------------------------------
public void testJoin_Objectarray() {
assertEquals(null, StringUtils.join(null));
assertEquals("", StringUtils.join(EMPTY_ARRAY_LIST));
assertEquals("", StringUtils.join(NULL_ARRAY_LIST));
assertEquals("abc", StringUtils.join(new String[] {"a", "b", "c"}));
assertEquals("a", StringUtils.join(new String[] {null, "a", ""}));
assertEquals("foo", StringUtils.join(MIXED_ARRAY_LIST));
assertEquals("foo2", StringUtils.join(MIXED_TYPE_LIST));
}
public void testJoin_ArrayChar() {
assertEquals(null, StringUtils.join((Object[]) null, ','));
assertEquals(TEXT_LIST_CHAR, StringUtils.join(ARRAY_LIST, SEPARATOR_CHAR));
assertEquals("", StringUtils.join(EMPTY_ARRAY_LIST, SEPARATOR_CHAR));
assertEquals(";;foo", StringUtils.join(MIXED_ARRAY_LIST, SEPARATOR_CHAR));
assertEquals("foo;2", StringUtils.join(MIXED_TYPE_LIST, SEPARATOR_CHAR));
assertEquals("/", StringUtils.join(MIXED_ARRAY_LIST, '/', 0, MIXED_ARRAY_LIST.length-1));
assertEquals("foo", StringUtils.join(MIXED_TYPE_LIST, '/', 0, 1));
assertEquals("foo/2", StringUtils.join(MIXED_TYPE_LIST, '/', 0, 2));
assertEquals("2", StringUtils.join(MIXED_TYPE_LIST, '/', 1, 2));
assertEquals("", StringUtils.join(MIXED_TYPE_LIST, '/', 2, 1));
}
public void testJoin_ArrayString() {
assertEquals(null, StringUtils.join((Object[]) null, null));
assertEquals(TEXT_LIST_NOSEP, StringUtils.join(ARRAY_LIST, null));
assertEquals(TEXT_LIST_NOSEP, StringUtils.join(ARRAY_LIST, ""));
assertEquals("", StringUtils.join(NULL_ARRAY_LIST, null));
assertEquals("", StringUtils.join(EMPTY_ARRAY_LIST, null));
assertEquals("", StringUtils.join(EMPTY_ARRAY_LIST, ""));
assertEquals("", StringUtils.join(EMPTY_ARRAY_LIST, SEPARATOR));
assertEquals(TEXT_LIST, StringUtils.join(ARRAY_LIST, SEPARATOR));
assertEquals(",,foo", StringUtils.join(MIXED_ARRAY_LIST, SEPARATOR));
assertEquals("foo,2", StringUtils.join(MIXED_TYPE_LIST, SEPARATOR));
assertEquals("/", StringUtils.join(MIXED_ARRAY_LIST, "/", 0, MIXED_ARRAY_LIST.length-1));
assertEquals("", StringUtils.join(MIXED_ARRAY_LIST, "", 0, MIXED_ARRAY_LIST.length-1));
assertEquals("foo", StringUtils.join(MIXED_TYPE_LIST, "/", 0, 1));
assertEquals("foo/2", StringUtils.join(MIXED_TYPE_LIST, "/", 0, 2));
assertEquals("2", StringUtils.join(MIXED_TYPE_LIST, "/", 1, 2));
assertEquals("", StringUtils.join(MIXED_TYPE_LIST, "/", 2, 1));
}
public void testJoin_IteratorChar() {
assertEquals(null, StringUtils.join((Iterator<?>) null, ','));
assertEquals(TEXT_LIST_CHAR, StringUtils.join(Arrays.asList(ARRAY_LIST).iterator(), SEPARATOR_CHAR));
assertEquals("", StringUtils.join(Arrays.asList(NULL_ARRAY_LIST).iterator(), SEPARATOR_CHAR));
assertEquals("", StringUtils.join(Arrays.asList(EMPTY_ARRAY_LIST).iterator(), SEPARATOR_CHAR));
assertEquals("foo", StringUtils.join(Collections.singleton("foo").iterator(), 'x'));
}
public void testJoin_IteratorString() {
assertEquals(null, StringUtils.join((Iterator<?>) null, null));
assertEquals(TEXT_LIST_NOSEP, StringUtils.join(Arrays.asList(ARRAY_LIST).iterator(), null));
assertEquals(TEXT_LIST_NOSEP, StringUtils.join(Arrays.asList(ARRAY_LIST).iterator(), ""));
assertEquals("foo", StringUtils.join(Collections.singleton("foo").iterator(), "x"));
assertEquals("foo", StringUtils.join(Collections.singleton("foo").iterator(), null));
assertEquals("", StringUtils.join(Arrays.asList(NULL_ARRAY_LIST).iterator(), null));
assertEquals("", StringUtils.join(Arrays.asList(EMPTY_ARRAY_LIST).iterator(), null));
assertEquals("", StringUtils.join(Arrays.asList(EMPTY_ARRAY_LIST).iterator(), ""));
assertEquals("", StringUtils.join(Arrays.asList(EMPTY_ARRAY_LIST).iterator(), SEPARATOR));
assertEquals(TEXT_LIST, StringUtils.join(Arrays.asList(ARRAY_LIST).iterator(), SEPARATOR));
}
public void testJoin_IterableChar() {
assertEquals(null, StringUtils.join((Iterable<?>) null, ','));
assertEquals(TEXT_LIST_CHAR, StringUtils.join(Arrays.asList(ARRAY_LIST), SEPARATOR_CHAR));
assertEquals("", StringUtils.join(Arrays.asList(NULL_ARRAY_LIST), SEPARATOR_CHAR));
assertEquals("", StringUtils.join(Arrays.asList(EMPTY_ARRAY_LIST), SEPARATOR_CHAR));
assertEquals("foo", StringUtils.join(Collections.singleton("foo"), 'x'));
}
public void testJoin_IterableString() {
assertEquals(null, StringUtils.join((Iterable<?>) null, null));
assertEquals(TEXT_LIST_NOSEP, StringUtils.join(Arrays.asList(ARRAY_LIST), null));
assertEquals(TEXT_LIST_NOSEP, StringUtils.join(Arrays.asList(ARRAY_LIST), ""));
assertEquals("foo", StringUtils.join(Collections.singleton("foo"), "x"));
assertEquals("foo", StringUtils.join(Collections.singleton("foo"), null));
assertEquals("", StringUtils.join(Arrays.asList(NULL_ARRAY_LIST), null));
assertEquals("", StringUtils.join(Arrays.asList(EMPTY_ARRAY_LIST), null));
assertEquals("", StringUtils.join(Arrays.asList(EMPTY_ARRAY_LIST), ""));
assertEquals("", StringUtils.join(Arrays.asList(EMPTY_ARRAY_LIST), SEPARATOR));
assertEquals(TEXT_LIST, StringUtils.join(Arrays.asList(ARRAY_LIST), SEPARATOR));
}
public void testSplit_String() {
assertEquals(null, StringUtils.split(null));
assertEquals(0, StringUtils.split("").length);
String str = "a b .c";
String[] res = StringUtils.split(str);
assertEquals(3, res.length);
assertEquals("a", res[0]);
assertEquals("b", res[1]);
assertEquals(".c", res[2]);
str = " a ";
res = StringUtils.split(str);
assertEquals(1, res.length);
assertEquals("a", res[0]);
str = "a" + WHITESPACE + "b" + NON_WHITESPACE + "c";
res = StringUtils.split(str);
assertEquals(2, res.length);
assertEquals("a", res[0]);
assertEquals("b" + NON_WHITESPACE + "c", res[1]);
}
public void testSplit_StringChar() {
assertEquals(null, StringUtils.split(null, '.'));
assertEquals(0, StringUtils.split("", '.').length);
String str = "a.b.. c";
String[] res = StringUtils.split(str, '.');
assertEquals(3, res.length);
assertEquals("a", res[0]);
assertEquals("b", res[1]);
assertEquals(" c", res[2]);
str = ".a.";
res = StringUtils.split(str, '.');
assertEquals(1, res.length);
assertEquals("a", res[0]);
str = "a b c";
res = StringUtils.split(str,' ');
assertEquals(3, res.length);
assertEquals("a", res[0]);
assertEquals("b", res[1]);
assertEquals("c", res[2]);
}
public void testSplit_StringString_StringStringInt() {
assertEquals(null, StringUtils.split(null, "."));
assertEquals(null, StringUtils.split(null, ".", 3));
assertEquals(0, StringUtils.split("", ".").length);
assertEquals(0, StringUtils.split("", ".", 3).length);
innerTestSplit('.', ".", ' ');
innerTestSplit('.', ".", ',');
innerTestSplit('.', ".,", 'x');
for (int i = 0; i < WHITESPACE.length(); i++) {
for (int j = 0; j < NON_WHITESPACE.length(); j++) {
innerTestSplit(WHITESPACE.charAt(i), null, NON_WHITESPACE.charAt(j));
innerTestSplit(WHITESPACE.charAt(i), String.valueOf(WHITESPACE.charAt(i)), NON_WHITESPACE.charAt(j));
}
}
String[] results = null;
String[] expectedResults = {"ab", "de fg"};
results = StringUtils.split("ab de fg", null, 2);
assertEquals(expectedResults.length, results.length);
for (int i = 0; i < expectedResults.length; i++) {
assertEquals(expectedResults[i], results[i]);
}
String[] expectedResults2 = {"ab", "cd:ef"};
results = StringUtils.split("ab:cd:ef",":", 2);
assertEquals(expectedResults2.length, results.length);
for (int i = 0; i < expectedResults2.length; i++) {
assertEquals(expectedResults2[i], results[i]);
}
}
private void innerTestSplit(char separator, String sepStr, char noMatch) {
String msg = "Failed on separator hex(" + Integer.toHexString(separator) +
"), noMatch hex(" + Integer.toHexString(noMatch) + "), sepStr(" + sepStr + ")";
final String str = "a" + separator + "b" + separator + separator + noMatch + "c";
String[] res;
// (str, sepStr)
res = StringUtils.split(str, sepStr);
assertEquals(msg, 3, res.length);
assertEquals(msg, "a", res[0]);
assertEquals(msg, "b", res[1]);
assertEquals(msg, noMatch + "c", res[2]);
final String str2 = separator + "a" + separator;
res = StringUtils.split(str2, sepStr);
assertEquals(msg, 1, res.length);
assertEquals(msg, "a", res[0]);
res = StringUtils.split(str, sepStr, -1);
assertEquals(msg, 3, res.length);
assertEquals(msg, "a", res[0]);
assertEquals(msg, "b", res[1]);
assertEquals(msg, noMatch + "c", res[2]);
res = StringUtils.split(str, sepStr, 0);
assertEquals(msg, 3, res.length);
assertEquals(msg, "a", res[0]);
assertEquals(msg, "b", res[1]);
assertEquals(msg, noMatch + "c", res[2]);
res = StringUtils.split(str, sepStr, 1);
assertEquals(msg, 1, res.length);
assertEquals(msg, str, res[0]);
res = StringUtils.split(str, sepStr, 2);
assertEquals(msg, 2, res.length);
assertEquals(msg, "a", res[0]);
assertEquals(msg, str.substring(2), res[1]);
}
public void testSplitByWholeString_StringStringBoolean() {
assertEquals( null, StringUtils.splitByWholeSeparator( null, "." ) ) ;
assertEquals( 0, StringUtils.splitByWholeSeparator( "", "." ).length ) ;
String stringToSplitOnNulls = "ab de fg" ;
String[] splitOnNullExpectedResults = { "ab", "de", "fg" } ;
String[] splitOnNullResults = StringUtils.splitByWholeSeparator( stringToSplitOnNulls, null ) ;
assertEquals( splitOnNullExpectedResults.length, splitOnNullResults.length ) ;
for ( int i = 0 ; i < splitOnNullExpectedResults.length ; i+= 1 ) {
assertEquals( splitOnNullExpectedResults[i], splitOnNullResults[i] ) ;
}
String stringToSplitOnCharactersAndString = "abstemiouslyaeiouyabstemiously" ;
String[] splitOnStringExpectedResults = { "abstemiously", "abstemiously" } ;
String[] splitOnStringResults = StringUtils.splitByWholeSeparator( stringToSplitOnCharactersAndString, "aeiouy" ) ;
assertEquals( splitOnStringExpectedResults.length, splitOnStringResults.length ) ;
for ( int i = 0 ; i < splitOnStringExpectedResults.length ; i+= 1 ) {
assertEquals( splitOnStringExpectedResults[i], splitOnStringResults[i] ) ;
}
String[] splitWithMultipleSeparatorExpectedResults = {"ab", "cd", "ef"};
String[] splitWithMultipleSeparator = StringUtils.splitByWholeSeparator("ab:cd::ef", ":");
assertEquals( splitWithMultipleSeparatorExpectedResults.length, splitWithMultipleSeparator.length );
for( int i = 0; i < splitWithMultipleSeparatorExpectedResults.length ; i++ ) {
assertEquals( splitWithMultipleSeparatorExpectedResults[i], splitWithMultipleSeparator[i] ) ;
}
}
public void testSplitByWholeString_StringStringBooleanInt() {
assertEquals( null, StringUtils.splitByWholeSeparator( null, ".", 3 ) ) ;
assertEquals( 0, StringUtils.splitByWholeSeparator( "", ".", 3 ).length ) ;
String stringToSplitOnNulls = "ab de fg" ;
String[] splitOnNullExpectedResults = { "ab", "de fg" } ;
//String[] splitOnNullExpectedResults = { "ab", "de" } ;
String[] splitOnNullResults = StringUtils.splitByWholeSeparator( stringToSplitOnNulls, null, 2 ) ;
assertEquals( splitOnNullExpectedResults.length, splitOnNullResults.length ) ;
for ( int i = 0 ; i < splitOnNullExpectedResults.length ; i+= 1 ) {
assertEquals( splitOnNullExpectedResults[i], splitOnNullResults[i] ) ;
}
String stringToSplitOnCharactersAndString = "abstemiouslyaeiouyabstemiouslyaeiouyabstemiously" ;
String[] splitOnStringExpectedResults = { "abstemiously", "abstemiouslyaeiouyabstemiously" } ;
//String[] splitOnStringExpectedResults = { "abstemiously", "abstemiously" } ;
String[] splitOnStringResults = StringUtils.splitByWholeSeparator( stringToSplitOnCharactersAndString, "aeiouy", 2 ) ;
assertEquals( splitOnStringExpectedResults.length, splitOnStringResults.length ) ;
for ( int i = 0 ; i < splitOnStringExpectedResults.length ; i++ ) {
assertEquals( splitOnStringExpectedResults[i], splitOnStringResults[i] ) ;
}
}
public void testSplitByWholeSeparatorPreserveAllTokens_StringStringInt() {
assertEquals( null, StringUtils.splitByWholeSeparatorPreserveAllTokens( null, ".", -1 ) ) ;
assertEquals( 0, StringUtils.splitByWholeSeparatorPreserveAllTokens( "", ".", -1 ).length ) ;
// test whitespace
String input = "ab de fg" ;
String[] expected = new String[] { "ab", "", "", "de", "fg" } ;
String[] actual = StringUtils.splitByWholeSeparatorPreserveAllTokens( input, null, -1 ) ;
assertEquals( expected.length, actual.length ) ;
for ( int i = 0 ; i < actual.length ; i+= 1 ) {
assertEquals( expected[i], actual[i] );
}
// test delimiter singlechar
input = "1::2:::3::::4";
expected = new String[] { "1", "", "2", "", "", "3", "", "", "", "4" };
actual = StringUtils.splitByWholeSeparatorPreserveAllTokens( input, ":", -1 ) ;
assertEquals( expected.length, actual.length ) ;
for ( int i = 0 ; i < actual.length ; i+= 1 ) {
assertEquals( expected[i], actual[i] );
}
// test delimiter multichar
input = "1::2:::3::::4";
expected = new String[] { "1", "2", ":3", "", "4" };
actual = StringUtils.splitByWholeSeparatorPreserveAllTokens( input, "::", -1 ) ;
assertEquals( expected.length, actual.length ) ;
for ( int i = 0 ; i < actual.length ; i+= 1 ) {
assertEquals( expected[i], actual[i] );
}
// test delimiter char with max
input = "1::2::3:4";
expected = new String[] { "1", "", "2", ":3:4" };
actual = StringUtils.splitByWholeSeparatorPreserveAllTokens( input, ":", 4 ) ;
assertEquals( expected.length, actual.length ) ;
for ( int i = 0 ; i < actual.length ; i+= 1 ) {
assertEquals( expected[i], actual[i] );
}
}
public void testSplitPreserveAllTokens_String() {
assertEquals(null, StringUtils.splitPreserveAllTokens(null));
assertEquals(0, StringUtils.splitPreserveAllTokens("").length);
String str = "abc def";
String[] res = StringUtils.splitPreserveAllTokens(str);
assertEquals(2, res.length);
assertEquals("abc", res[0]);
assertEquals("def", res[1]);
str = "abc def";
res = StringUtils.splitPreserveAllTokens(str);
assertEquals(3, res.length);
assertEquals("abc", res[0]);
assertEquals("", res[1]);
assertEquals("def", res[2]);
str = " abc ";
res = StringUtils.splitPreserveAllTokens(str);
assertEquals(3, res.length);
assertEquals("", res[0]);
assertEquals("abc", res[1]);
assertEquals("", res[2]);
str = "a b .c";
res = StringUtils.splitPreserveAllTokens(str);
assertEquals(3, res.length);
assertEquals("a", res[0]);
assertEquals("b", res[1]);
assertEquals(".c", res[2]);
str = " a b .c";
res = StringUtils.splitPreserveAllTokens(str);
assertEquals(4, res.length);
assertEquals("", res[0]);
assertEquals("a", res[1]);
assertEquals("b", res[2]);
assertEquals(".c", res[3]);
str = "a b .c";
res = StringUtils.splitPreserveAllTokens(str);
assertEquals(5, res.length);
assertEquals("a", res[0]);
assertEquals("", res[1]);
assertEquals("b", res[2]);
assertEquals("", res[3]);
assertEquals(".c", res[4]);
str = " a ";
res = StringUtils.splitPreserveAllTokens(str);
assertEquals(4, res.length);
assertEquals("", res[0]);
assertEquals("a", res[1]);
assertEquals("", res[2]);
assertEquals("", res[3]);
str = " a b";
res = StringUtils.splitPreserveAllTokens(str);
assertEquals(4, res.length);
assertEquals("", res[0]);
assertEquals("a", res[1]);
assertEquals("", res[2]);
assertEquals("b", res[3]);
str = "a" + WHITESPACE + "b" + NON_WHITESPACE + "c";
res = StringUtils.splitPreserveAllTokens(str);
assertEquals(WHITESPACE.length() + 1, res.length);
assertEquals("a", res[0]);
for(int i = 1; i < WHITESPACE.length()-1; i++)
{
assertEquals("", res[i]);
}
assertEquals("b" + NON_WHITESPACE + "c", res[WHITESPACE.length()]);
}
public void testSplitPreserveAllTokens_StringChar() {
assertEquals(null, StringUtils.splitPreserveAllTokens(null, '.'));
assertEquals(0, StringUtils.splitPreserveAllTokens("", '.').length);
String str = "a.b. c";
String[] res = StringUtils.splitPreserveAllTokens(str, '.');
assertEquals(3, res.length);
assertEquals("a", res[0]);
assertEquals("b", res[1]);
assertEquals(" c", res[2]);
str = "a.b.. c";
res = StringUtils.splitPreserveAllTokens(str, '.');
assertEquals(4, res.length);
assertEquals("a", res[0]);
assertEquals("b", res[1]);
assertEquals("", res[2]);
assertEquals(" c", res[3]);
str = ".a.";
res = StringUtils.splitPreserveAllTokens(str, '.');
assertEquals(3, res.length);
assertEquals("", res[0]);
assertEquals("a", res[1]);
assertEquals("", res[2]);
str = ".a..";
res = StringUtils.splitPreserveAllTokens(str, '.');
assertEquals(4, res.length);
assertEquals("", res[0]);
assertEquals("a", res[1]);
assertEquals("", res[2]);
assertEquals("", res[3]);
str = "..a.";
res = StringUtils.splitPreserveAllTokens(str, '.');
assertEquals(4, res.length);
assertEquals("", res[0]);
assertEquals("", res[1]);
assertEquals("a", res[2]);
assertEquals("", res[3]);
str = "..a";
res = StringUtils.splitPreserveAllTokens(str, '.');
assertEquals(3, res.length);
assertEquals("", res[0]);
assertEquals("", res[1]);
assertEquals("a", res[2]);
str = "a b c";
res = StringUtils.splitPreserveAllTokens(str,' ');
assertEquals(3, res.length);
assertEquals("a", res[0]);
assertEquals("b", res[1]);
assertEquals("c", res[2]);
str = "a b c";
res = StringUtils.splitPreserveAllTokens(str,' ');
assertEquals(5, res.length);
assertEquals("a", res[0]);
assertEquals("", res[1]);
assertEquals("b", res[2]);
assertEquals("", res[3]);
assertEquals("c", res[4]);
str = " a b c";
res = StringUtils.splitPreserveAllTokens(str,' ');
assertEquals(4, res.length);
assertEquals("", res[0]);
assertEquals("a", res[1]);
assertEquals("b", res[2]);
assertEquals("c", res[3]);
str = " a b c";
res = StringUtils.splitPreserveAllTokens(str,' ');
assertEquals(5, res.length);
assertEquals("", res[0]);
assertEquals("", res[1]);
assertEquals("a", res[2]);
assertEquals("b", res[3]);
assertEquals("c", res[4]);
str = "a b c ";
res = StringUtils.splitPreserveAllTokens(str,' ');
assertEquals(4, res.length);
assertEquals("a", res[0]);
assertEquals("b", res[1]);
assertEquals("c", res[2]);
assertEquals("", res[3]);
str = "a b c ";
res = StringUtils.splitPreserveAllTokens(str,' ');
assertEquals(5, res.length);
assertEquals("a", res[0]);
assertEquals("b", res[1]);
assertEquals("c", res[2]);
assertEquals("", res[3]);
assertEquals("", res[3]);
// Match example in javadoc
{
String[] results = null;
String[] expectedResults = {"a", "", "b", "c"};
results = StringUtils.splitPreserveAllTokens("a..b.c",'.');
assertEquals(expectedResults.length, results.length);
for (int i = 0; i < expectedResults.length; i++) {
assertEquals(expectedResults[i], results[i]);
}
}
}
public void testSplitPreserveAllTokens_StringString_StringStringInt() {
assertEquals(null, StringUtils.splitPreserveAllTokens(null, "."));
assertEquals(null, StringUtils.splitPreserveAllTokens(null, ".", 3));
assertEquals(0, StringUtils.splitPreserveAllTokens("", ".").length);
assertEquals(0, StringUtils.splitPreserveAllTokens("", ".", 3).length);
innerTestSplitPreserveAllTokens('.', ".", ' ');
innerTestSplitPreserveAllTokens('.', ".", ',');
innerTestSplitPreserveAllTokens('.', ".,", 'x');
for (int i = 0; i < WHITESPACE.length(); i++) {
for (int j = 0; j < NON_WHITESPACE.length(); j++) {
innerTestSplitPreserveAllTokens(WHITESPACE.charAt(i), null, NON_WHITESPACE.charAt(j));
innerTestSplitPreserveAllTokens(WHITESPACE.charAt(i), String.valueOf(WHITESPACE.charAt(i)), NON_WHITESPACE.charAt(j));
}
}
{
String[] results = null;
String[] expectedResults = {"ab", "de fg"};
results = StringUtils.splitPreserveAllTokens("ab de fg", null, 2);
assertEquals(expectedResults.length, results.length);
for (int i = 0; i < expectedResults.length; i++) {
assertEquals(expectedResults[i], results[i]);
}
}
{
String[] results = null;
String[] expectedResults = {"ab", " de fg"};
results = StringUtils.splitPreserveAllTokens("ab de fg", null, 2);
assertEquals(expectedResults.length, results.length);
for (int i = 0; i < expectedResults.length; i++) {
assertEquals(expectedResults[i], results[i]);
}
}
{
String[] results = null;
String[] expectedResults = {"ab", "::de:fg"};
results = StringUtils.splitPreserveAllTokens("ab:::de:fg", ":", 2);
assertEquals(expectedResults.length, results.length);
for (int i = 0; i < expectedResults.length; i++) {
assertEquals(expectedResults[i], results[i]);
}
}
{
String[] results = null;
String[] expectedResults = {"ab", "", " de fg"};
results = StringUtils.splitPreserveAllTokens("ab de fg", null, 3);
assertEquals(expectedResults.length, results.length);
for (int i = 0; i < expectedResults.length; i++) {
assertEquals(expectedResults[i], results[i]);
}
}
{
String[] results = null;
String[] expectedResults = {"ab", "", "", "de fg"};
results = StringUtils.splitPreserveAllTokens("ab de fg", null, 4);
assertEquals(expectedResults.length, results.length);
for (int i = 0; i < expectedResults.length; i++) {
assertEquals(expectedResults[i], results[i]);
}
}
{
String[] expectedResults = {"ab", "cd:ef"};
String[] results = null;
results = StringUtils.splitPreserveAllTokens("ab:cd:ef",":", 2);
assertEquals(expectedResults.length, results.length);
for (int i = 0; i < expectedResults.length; i++) {
assertEquals(expectedResults[i], results[i]);
}
}
{
String[] results = null;
String[] expectedResults = {"ab", ":cd:ef"};
results = StringUtils.splitPreserveAllTokens("ab::cd:ef",":", 2);
assertEquals(expectedResults.length, results.length);
for (int i = 0; i < expectedResults.length; i++) {
assertEquals(expectedResults[i], results[i]);
}
}
{
String[] results = null;
String[] expectedResults = {"ab", "", ":cd:ef"};
results = StringUtils.splitPreserveAllTokens("ab:::cd:ef",":", 3);
assertEquals(expectedResults.length, results.length);
for (int i = 0; i < expectedResults.length; i++) {
assertEquals(expectedResults[i], results[i]);
}
}
{
String[] results = null;
String[] expectedResults = {"ab", "", "", "cd:ef"};
results = StringUtils.splitPreserveAllTokens("ab:::cd:ef",":", 4);
assertEquals(expectedResults.length, results.length);
for (int i = 0; i < expectedResults.length; i++) {
assertEquals(expectedResults[i], results[i]);
}
}
{
String[] results = null;
String[] expectedResults = {"", "ab", "", "", "cd:ef"};
results = StringUtils.splitPreserveAllTokens(":ab:::cd:ef",":", 5);
assertEquals(expectedResults.length, results.length);
for (int i = 0; i < expectedResults.length; i++) {
assertEquals(expectedResults[i], results[i]);
}
}
{
String[] results = null;
String[] expectedResults = {"", "", "ab", "", "", "cd:ef"};
results = StringUtils.splitPreserveAllTokens("::ab:::cd:ef",":", 6);
assertEquals(expectedResults.length, results.length);
for (int i = 0; i < expectedResults.length; i++) {
assertEquals(expectedResults[i], results[i]);
}
}
}
private void innerTestSplitPreserveAllTokens(char separator, String sepStr, char noMatch) {
String msg = "Failed on separator hex(" + Integer.toHexString(separator) +
"), noMatch hex(" + Integer.toHexString(noMatch) + "), sepStr(" + sepStr + ")";
final String str = "a" + separator + "b" + separator + separator + noMatch + "c";
String[] res;
// (str, sepStr)
res = StringUtils.splitPreserveAllTokens(str, sepStr);
assertEquals(msg, 4, res.length);
assertEquals(msg, "a", res[0]);
assertEquals(msg, "b", res[1]);
assertEquals(msg, "", res[2]);
assertEquals(msg, noMatch + "c", res[3]);
final String str2 = separator + "a" + separator;
res = StringUtils.splitPreserveAllTokens(str2, sepStr);
assertEquals(msg, 3, res.length);
assertEquals(msg, "", res[0]);
assertEquals(msg, "a", res[1]);
assertEquals(msg, "", res[2]);
res = StringUtils.splitPreserveAllTokens(str, sepStr, -1);
assertEquals(msg, 4, res.length);
assertEquals(msg, "a", res[0]);
assertEquals(msg, "b", res[1]);
assertEquals(msg, "", res[2]);
assertEquals(msg, noMatch + "c", res[3]);
res = StringUtils.splitPreserveAllTokens(str, sepStr, 0);
assertEquals(msg, 4, res.length);
assertEquals(msg, "a", res[0]);
assertEquals(msg, "b", res[1]);
assertEquals(msg, "", res[2]);
assertEquals(msg, noMatch + "c", res[3]);
res = StringUtils.splitPreserveAllTokens(str, sepStr, 1);
assertEquals(msg, 1, res.length);
assertEquals(msg, str, res[0]);
res = StringUtils.splitPreserveAllTokens(str, sepStr, 2);
assertEquals(msg, 2, res.length);
assertEquals(msg, "a", res[0]);
assertEquals(msg, str.substring(2), res[1]);
}
public void testSplitByCharacterType() {
assertNull(StringUtils.splitByCharacterType(null));
assertEquals(0, StringUtils.splitByCharacterType("").length);
assertTrue(ArrayUtils.isEquals(new String[] { "ab", " ", "de", " ",
"fg" }, StringUtils.splitByCharacterType("ab de fg")));
assertTrue(ArrayUtils.isEquals(new String[] { "ab", " ", "de", " ",
"fg" }, StringUtils.splitByCharacterType("ab de fg")));
assertTrue(ArrayUtils.isEquals(new String[] { "ab", ":", "cd", ":",
"ef" }, StringUtils.splitByCharacterType("ab:cd:ef")));
assertTrue(ArrayUtils.isEquals(new String[] { "number", "5" },
StringUtils.splitByCharacterType("number5")));
assertTrue(ArrayUtils.isEquals(new String[] { "foo", "B", "ar" },
StringUtils.splitByCharacterType("fooBar")));
assertTrue(ArrayUtils.isEquals(new String[] { "foo", "200", "B", "ar" },
StringUtils.splitByCharacterType("foo200Bar")));
assertTrue(ArrayUtils.isEquals(new String[] { "ASFR", "ules" },
StringUtils.splitByCharacterType("ASFRules")));
}
public void testSplitByCharacterTypeCamelCase() {
assertNull(StringUtils.splitByCharacterTypeCamelCase(null));
assertEquals(0, StringUtils.splitByCharacterTypeCamelCase("").length);
assertTrue(ArrayUtils.isEquals(new String[] { "ab", " ", "de", " ",
"fg" }, StringUtils.splitByCharacterTypeCamelCase("ab de fg")));
assertTrue(ArrayUtils.isEquals(new String[] { "ab", " ", "de", " ",
"fg" }, StringUtils.splitByCharacterTypeCamelCase("ab de fg")));
assertTrue(ArrayUtils.isEquals(new String[] { "ab", ":", "cd", ":",
"ef" }, StringUtils.splitByCharacterTypeCamelCase("ab:cd:ef")));
assertTrue(ArrayUtils.isEquals(new String[] { "number", "5" },
StringUtils.splitByCharacterTypeCamelCase("number5")));
assertTrue(ArrayUtils.isEquals(new String[] { "foo", "Bar" },
StringUtils.splitByCharacterTypeCamelCase("fooBar")));
assertTrue(ArrayUtils.isEquals(new String[] { "foo", "200", "Bar" },
StringUtils.splitByCharacterTypeCamelCase("foo200Bar")));
assertTrue(ArrayUtils.isEquals(new String[] { "ASF", "Rules" },
StringUtils.splitByCharacterTypeCamelCase("ASFRules")));
}
public void testDeleteWhitespace_String() {
assertEquals(null, StringUtils.deleteWhitespace(null));
assertEquals("", StringUtils.deleteWhitespace(""));
assertEquals("", StringUtils.deleteWhitespace(" \u000C \t\t\u001F\n\n \u000B "));
assertEquals("", StringUtils.deleteWhitespace(StringUtilsTest.WHITESPACE));
assertEquals(StringUtilsTest.NON_WHITESPACE, StringUtils.deleteWhitespace(StringUtilsTest.NON_WHITESPACE));
// Note: u-2007 and u-000A both cause problems in the source code
// it should ignore 2007 but delete 000A
assertEquals("\u00A0\u202F", StringUtils.deleteWhitespace(" \u00A0 \t\t\n\n \u202F "));
assertEquals("\u00A0\u202F", StringUtils.deleteWhitespace("\u00A0\u202F"));
assertEquals("test", StringUtils.deleteWhitespace("\u000Bt \t\n\u0009e\rs\n\n \tt"));
}
public void testReplace_StringStringString() {
assertEquals(null, StringUtils.replace(null, null, null));
assertEquals(null, StringUtils.replace(null, null, "any"));
assertEquals(null, StringUtils.replace(null, "any", null));
assertEquals(null, StringUtils.replace(null, "any", "any"));
assertEquals("", StringUtils.replace("", null, null));
assertEquals("", StringUtils.replace("", null, "any"));
assertEquals("", StringUtils.replace("", "any", null));
assertEquals("", StringUtils.replace("", "any", "any"));
assertEquals("FOO", StringUtils.replace("FOO", "", "any"));
assertEquals("FOO", StringUtils.replace("FOO", null, "any"));
assertEquals("FOO", StringUtils.replace("FOO", "F", null));
assertEquals("FOO", StringUtils.replace("FOO", null, null));
assertEquals("", StringUtils.replace("foofoofoo", "foo", ""));
assertEquals("barbarbar", StringUtils.replace("foofoofoo", "foo", "bar"));
assertEquals("farfarfar", StringUtils.replace("foofoofoo", "oo", "ar"));
}
public void testReplace_StringStringStringInt() {
assertEquals(null, StringUtils.replace(null, null, null, 2));
assertEquals(null, StringUtils.replace(null, null, "any", 2));
assertEquals(null, StringUtils.replace(null, "any", null, 2));
assertEquals(null, StringUtils.replace(null, "any", "any", 2));
assertEquals("", StringUtils.replace("", null, null, 2));
assertEquals("", StringUtils.replace("", null, "any", 2));
assertEquals("", StringUtils.replace("", "any", null, 2));
assertEquals("", StringUtils.replace("", "any", "any", 2));
String str = new String(new char[] {'o', 'o', 'f', 'o', 'o'});
assertSame(str, StringUtils.replace(str, "x", "", -1));
assertEquals("f", StringUtils.replace("oofoo", "o", "", -1));
assertEquals("oofoo", StringUtils.replace("oofoo", "o", "", 0));
assertEquals("ofoo", StringUtils.replace("oofoo", "o", "", 1));
assertEquals("foo", StringUtils.replace("oofoo", "o", "", 2));
assertEquals("fo", StringUtils.replace("oofoo", "o", "", 3));
assertEquals("f", StringUtils.replace("oofoo", "o", "", 4));
assertEquals("f", StringUtils.replace("oofoo", "o", "", -5));
assertEquals("f", StringUtils.replace("oofoo", "o", "", 1000));
}
public void testReplaceOnce_StringStringString() {
assertEquals(null, StringUtils.replaceOnce(null, null, null));
assertEquals(null, StringUtils.replaceOnce(null, null, "any"));
assertEquals(null, StringUtils.replaceOnce(null, "any", null));
assertEquals(null, StringUtils.replaceOnce(null, "any", "any"));
assertEquals("", StringUtils.replaceOnce("", null, null));
assertEquals("", StringUtils.replaceOnce("", null, "any"));
assertEquals("", StringUtils.replaceOnce("", "any", null));
assertEquals("", StringUtils.replaceOnce("", "any", "any"));
assertEquals("FOO", StringUtils.replaceOnce("FOO", "", "any"));
assertEquals("FOO", StringUtils.replaceOnce("FOO", null, "any"));
assertEquals("FOO", StringUtils.replaceOnce("FOO", "F", null));
assertEquals("FOO", StringUtils.replaceOnce("FOO", null, null));
assertEquals("foofoo", StringUtils.replaceOnce("foofoofoo", "foo", ""));
}
/**
* Test method for 'StringUtils.replaceEach(String, String[], String[])'
*/
public void testReplace_StringStringArrayStringArray() {
//JAVADOC TESTS START
assertNull(StringUtils.replaceEach(null, new String[]{"a"}, new String[]{"b"}));
assertEquals(StringUtils.replaceEach("", new String[]{"a"}, new String[]{"b"}),"");
assertEquals(StringUtils.replaceEach("aba", null, null),"aba");
assertEquals(StringUtils.replaceEach("aba", new String[0], null),"aba");
assertEquals(StringUtils.replaceEach("aba", null, new String[0]),"aba");
assertEquals(StringUtils.replaceEach("aba", new String[]{"a"}, null),"aba");
assertEquals(StringUtils.replaceEach("aba", new String[]{"a"}, new String[]{""}),"b");
assertEquals(StringUtils.replaceEach("aba", new String[]{null}, new String[]{"a"}),"aba");
assertEquals(StringUtils.replaceEach("abcde", new String[]{"ab", "d"}, new String[]{"w", "t"}),"wcte");
assertEquals(StringUtils.replaceEach("abcde", new String[]{"ab", "d"}, new String[]{"d", "t"}),"dcte");
//JAVADOC TESTS END
assertEquals("bcc", StringUtils.replaceEach("abc", new String[]{"a", "b"}, new String[]{"b", "c"}));
assertEquals("q651.506bera", StringUtils.replaceEach("d216.102oren",
new String[]{"a", "b", "c", "d", "e", "f", "g", "h", "i", "j", "k", "l", "m", "n",
"o", "p", "q", "r", "s", "t", "u", "v", "w", "x", "y", "z", "A", "B", "C", "D",
"E", "F", "G", "H", "I", "J", "K", "L", "M", "N", "O", "P", "Q", "R", "S", "T",
"U", "V", "W", "X", "Y", "Z", "1", "2", "3", "4", "5", "6", "7", "8", "9"},
new String[]{"n", "o", "p", "q", "r", "s", "t", "u", "v", "w", "x", "y", "z", "a",
"b", "c", "d", "e", "f", "g", "h", "i", "j", "k", "l", "m", "N", "O", "P", "Q",
"R", "S", "T", "U", "V", "W", "X", "Y", "Z", "A", "B", "C", "D", "E", "F", "G",
"H", "I", "J", "K", "L", "M", "5", "6", "7", "8", "9", "1", "2", "3", "4"}));
// Test null safety inside arrays - LANG-552
assertEquals(StringUtils.replaceEach("aba", new String[]{"a"}, new String[]{null}),"aba");
assertEquals(StringUtils.replaceEach("aba", new String[]{"a", "b"}, new String[]{"c", null}),"cbc");
}
/**
* Test method for 'StringUtils.replaceEachRepeatedly(String, String[], String[])'
*/
public void testReplace_StringStringArrayStringArrayBoolean() {
//JAVADOC TESTS START
assertNull(StringUtils.replaceEachRepeatedly(null, new String[]{"a"}, new String[]{"b"}));
assertEquals(StringUtils.replaceEachRepeatedly("", new String[]{"a"}, new String[]{"b"}),"");
assertEquals(StringUtils.replaceEachRepeatedly("aba", null, null),"aba");
assertEquals(StringUtils.replaceEachRepeatedly("aba", new String[0], null),"aba");
assertEquals(StringUtils.replaceEachRepeatedly("aba", null, new String[0]),"aba");
assertEquals(StringUtils.replaceEachRepeatedly("aba", new String[0], null),"aba");
assertEquals(StringUtils.replaceEachRepeatedly("aba", new String[]{"a"}, new String[]{""}),"b");
assertEquals(StringUtils.replaceEachRepeatedly("aba", new String[]{null}, new String[]{"a"}),"aba");
assertEquals(StringUtils.replaceEachRepeatedly("abcde", new String[]{"ab", "d"}, new String[]{"w", "t"}),"wcte");
assertEquals(StringUtils.replaceEachRepeatedly("abcde", new String[]{"ab", "d"}, new String[]{"d", "t"}),"tcte");
try {
StringUtils.replaceEachRepeatedly("abcde", new String[]{"ab", "d"}, new String[]{"d", "ab"});
fail("Should be a circular reference");
} catch (IllegalStateException e) {}
//JAVADOC TESTS END
}
public void testReplaceChars_StringCharChar() {
assertEquals(null, StringUtils.replaceChars(null, 'b', 'z'));
assertEquals("", StringUtils.replaceChars("", 'b', 'z'));
assertEquals("azcza", StringUtils.replaceChars("abcba", 'b', 'z'));
assertEquals("abcba", StringUtils.replaceChars("abcba", 'x', 'z'));
}
public void testReplaceChars_StringStringString() {
assertEquals(null, StringUtils.replaceChars(null, null, null));
assertEquals(null, StringUtils.replaceChars(null, "", null));
assertEquals(null, StringUtils.replaceChars(null, "a", null));
assertEquals(null, StringUtils.replaceChars(null, null, ""));
assertEquals(null, StringUtils.replaceChars(null, null, "x"));
assertEquals("", StringUtils.replaceChars("", null, null));
assertEquals("", StringUtils.replaceChars("", "", null));
assertEquals("", StringUtils.replaceChars("", "a", null));
assertEquals("", StringUtils.replaceChars("", null, ""));
assertEquals("", StringUtils.replaceChars("", null, "x"));
assertEquals("abc", StringUtils.replaceChars("abc", null, null));
assertEquals("abc", StringUtils.replaceChars("abc", null, ""));
assertEquals("abc", StringUtils.replaceChars("abc", null, "x"));
assertEquals("abc", StringUtils.replaceChars("abc", "", null));
assertEquals("abc", StringUtils.replaceChars("abc", "", ""));
assertEquals("abc", StringUtils.replaceChars("abc", "", "x"));
assertEquals("ac", StringUtils.replaceChars("abc", "b", null));
assertEquals("ac", StringUtils.replaceChars("abc", "b", ""));
assertEquals("axc", StringUtils.replaceChars("abc", "b", "x"));
assertEquals("ayzya", StringUtils.replaceChars("abcba", "bc", "yz"));
assertEquals("ayya", StringUtils.replaceChars("abcba", "bc", "y"));
assertEquals("ayzya", StringUtils.replaceChars("abcba", "bc", "yzx"));
assertEquals("abcba", StringUtils.replaceChars("abcba", "z", "w"));
assertSame("abcba", StringUtils.replaceChars("abcba", "z", "w"));
// Javadoc examples:
assertEquals("jelly", StringUtils.replaceChars("hello", "ho", "jy"));
assertEquals("ayzya", StringUtils.replaceChars("abcba", "bc", "yz"));
assertEquals("ayya", StringUtils.replaceChars("abcba", "bc", "y"));
assertEquals("ayzya", StringUtils.replaceChars("abcba", "bc", "yzx"));
// From http://issues.apache.org/bugzilla/show_bug.cgi?id=25454
assertEquals("bcc", StringUtils.replaceChars("abc", "ab", "bc"));
assertEquals("q651.506bera", StringUtils.replaceChars("d216.102oren",
"abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ123456789",
"nopqrstuvwxyzabcdefghijklmNOPQRSTUVWXYZABCDEFGHIJKLM567891234"));
}
public void testOverlay_StringStringIntInt() {
assertEquals(null, StringUtils.overlay(null, null, 2, 4));
assertEquals(null, StringUtils.overlay(null, null, -2, -4));
assertEquals("", StringUtils.overlay("", null, 0, 0));
assertEquals("", StringUtils.overlay("", "", 0, 0));
assertEquals("zzzz", StringUtils.overlay("", "zzzz", 0, 0));
assertEquals("zzzz", StringUtils.overlay("", "zzzz", 2, 4));
assertEquals("zzzz", StringUtils.overlay("", "zzzz", -2, -4));
assertEquals("abef", StringUtils.overlay("abcdef", null, 2, 4));
assertEquals("abef", StringUtils.overlay("abcdef", null, 4, 2));
assertEquals("abef", StringUtils.overlay("abcdef", "", 2, 4));
assertEquals("abef", StringUtils.overlay("abcdef", "", 4, 2));
assertEquals("abzzzzef", StringUtils.overlay("abcdef", "zzzz", 2, 4));
assertEquals("abzzzzef", StringUtils.overlay("abcdef", "zzzz", 4, 2));
assertEquals("zzzzef", StringUtils.overlay("abcdef", "zzzz", -1, 4));
assertEquals("zzzzef", StringUtils.overlay("abcdef", "zzzz", 4, -1));
assertEquals("zzzzabcdef", StringUtils.overlay("abcdef", "zzzz", -2, -1));
assertEquals("zzzzabcdef", StringUtils.overlay("abcdef", "zzzz", -1, -2));
assertEquals("abcdzzzz", StringUtils.overlay("abcdef", "zzzz", 4, 10));
assertEquals("abcdzzzz", StringUtils.overlay("abcdef", "zzzz", 10, 4));
assertEquals("abcdefzzzz", StringUtils.overlay("abcdef", "zzzz", 8, 10));
assertEquals("abcdefzzzz", StringUtils.overlay("abcdef", "zzzz", 10, 8));
}
public void testRepeat_StringInt() {
assertEquals(null, StringUtils.repeat(null, 2));
assertEquals("", StringUtils.repeat("ab", 0));
assertEquals("", StringUtils.repeat("", 3));
assertEquals("aaa", StringUtils.repeat("a", 3));
assertEquals("ababab", StringUtils.repeat("ab", 3));
assertEquals("abcabcabc", StringUtils.repeat("abc", 3));
String str = StringUtils.repeat("a", 10000); // bigger than pad limit
assertEquals(10000, str.length());
assertEquals(true, StringUtils.containsOnly(str, new char[] {'a'}));
}
public void testRepeat_StringStringInt() {
assertEquals(null, StringUtils.repeat(null, null, 2));
assertEquals(null, StringUtils.repeat(null, "x", 2));
assertEquals("", StringUtils.repeat("", null, 2));
assertEquals("", StringUtils.repeat("ab", "", 0));
assertEquals("", StringUtils.repeat("", "", 2));
assertEquals("xx", StringUtils.repeat("", "x", 3));
assertEquals("?, ?, ?", StringUtils.repeat("?", ", ", 3));
}
public void testChop() {
String[][] chopCases = {
{ FOO_UNCAP + "\r\n", FOO_UNCAP } ,
{ FOO_UNCAP + "\n" , FOO_UNCAP } ,
{ FOO_UNCAP + "\r", FOO_UNCAP },
{ FOO_UNCAP + " \r", FOO_UNCAP + " " },
{ "foo", "fo"},
{ "foo\nfoo", "foo\nfo" },
{ "\n", "" },
{ "\r", "" },
{ "\r\n", "" },
{ null, null },
{ "", "" },
{ "a", "" },
};
for (int i = 0; i < chopCases.length; i++) {
String original = chopCases[i][0];
String expectedResult = chopCases[i][1];
assertEquals("chop(String) failed",
expectedResult, StringUtils.chop(original));
}
}
public void testChomp() {
String[][] chompCases = {
{ FOO_UNCAP + "\r\n", FOO_UNCAP },
{ FOO_UNCAP + "\n" , FOO_UNCAP },
{ FOO_UNCAP + "\r", FOO_UNCAP },
{ FOO_UNCAP + " \r", FOO_UNCAP + " " },
{ FOO_UNCAP, FOO_UNCAP },
{ FOO_UNCAP + "\n\n", FOO_UNCAP + "\n"},
{ FOO_UNCAP + "\r\n\r\n", FOO_UNCAP + "\r\n" },
{ "foo\nfoo", "foo\nfoo" },
{ "foo\n\rfoo", "foo\n\rfoo" },
{ "\n", "" },
{ "\r", "" },
{ "a", "a" },
{ "\r\n", "" },
{ "", "" },
{ null, null },
{ FOO_UNCAP + "\n\r", FOO_UNCAP + "\n"}
};
for (int i = 0; i < chompCases.length; i++) {
String original = chompCases[i][0];
String expectedResult = chompCases[i][1];
assertEquals("chomp(String) failed",
expectedResult, StringUtils.chomp(original));
}
assertEquals("chomp(String, String) failed",
"foo", StringUtils.chomp("foobar", "bar"));
assertEquals("chomp(String, String) failed",
"foobar", StringUtils.chomp("foobar", "baz"));
assertEquals("chomp(String, String) failed",
"foo", StringUtils.chomp("foo", "foooo"));
assertEquals("chomp(String, String) failed",
"foobar", StringUtils.chomp("foobar", ""));
assertEquals("chomp(String, String) failed",
"foobar", StringUtils.chomp("foobar", null));
assertEquals("chomp(String, String) failed",
"", StringUtils.chomp("", "foo"));
assertEquals("chomp(String, String) failed",
"", StringUtils.chomp("", null));
assertEquals("chomp(String, String) failed",
"", StringUtils.chomp("", ""));
assertEquals("chomp(String, String) failed",
null, StringUtils.chomp(null, "foo"));
assertEquals("chomp(String, String) failed",
null, StringUtils.chomp(null, null));
assertEquals("chomp(String, String) failed",
null, StringUtils.chomp(null, ""));
assertEquals("chomp(String, String) failed",
"", StringUtils.chomp("foo", "foo"));
assertEquals("chomp(String, String) failed",
" ", StringUtils.chomp(" foo", "foo"));
assertEquals("chomp(String, String) failed",
"foo ", StringUtils.chomp("foo ", "foo"));
}
//-----------------------------------------------------------------------
public void testRightPad_StringInt() {
assertEquals(null, StringUtils.rightPad(null, 5));
assertEquals(" ", StringUtils.rightPad("", 5));
assertEquals("abc ", StringUtils.rightPad("abc", 5));
assertEquals("abc", StringUtils.rightPad("abc", 2));
assertEquals("abc", StringUtils.rightPad("abc", -1));
}
public void testRightPad_StringIntChar() {
assertEquals(null, StringUtils.rightPad(null, 5, ' '));
assertEquals(" ", StringUtils.rightPad("", 5, ' '));
assertEquals("abc ", StringUtils.rightPad("abc", 5, ' '));
assertEquals("abc", StringUtils.rightPad("abc", 2, ' '));
assertEquals("abc", StringUtils.rightPad("abc", -1, ' '));
assertEquals("abcxx", StringUtils.rightPad("abc", 5, 'x'));
String str = StringUtils.rightPad("aaa", 10000, 'a'); // bigger than pad length
assertEquals(10000, str.length());
assertEquals(true, StringUtils.containsOnly(str, new char[] {'a'}));
}
public void testRightPad_StringIntString() {
assertEquals(null, StringUtils.rightPad(null, 5, "-+"));
assertEquals(" ", StringUtils.rightPad("", 5, " "));
assertEquals(null, StringUtils.rightPad(null, 8, null));
assertEquals("abc-+-+", StringUtils.rightPad("abc", 7, "-+"));
assertEquals("abc-+~", StringUtils.rightPad("abc", 6, "-+~"));
assertEquals("abc-+", StringUtils.rightPad("abc", 5, "-+~"));
assertEquals("abc", StringUtils.rightPad("abc", 2, " "));
assertEquals("abc", StringUtils.rightPad("abc", -1, " "));
assertEquals("abc ", StringUtils.rightPad("abc", 5, null));
assertEquals("abc ", StringUtils.rightPad("abc", 5, ""));
}
//-----------------------------------------------------------------------
public void testLeftPad_StringInt() {
assertEquals(null, StringUtils.leftPad(null, 5));
assertEquals(" ", StringUtils.leftPad("", 5));
assertEquals(" abc", StringUtils.leftPad("abc", 5));
assertEquals("abc", StringUtils.leftPad("abc", 2));
}
public void testLeftPad_StringIntChar() {
assertEquals(null, StringUtils.leftPad(null, 5, ' '));
assertEquals(" ", StringUtils.leftPad("", 5, ' '));
assertEquals(" abc", StringUtils.leftPad("abc", 5, ' '));
assertEquals("xxabc", StringUtils.leftPad("abc", 5, 'x'));
assertEquals("\uffff\uffffabc", StringUtils.leftPad("abc", 5, '\uffff'));
assertEquals("abc", StringUtils.leftPad("abc", 2, ' '));
String str = StringUtils.leftPad("aaa", 10000, 'a'); // bigger than pad length
assertEquals(10000, str.length());
assertEquals(true, StringUtils.containsOnly(str, new char[] {'a'}));
}
public void testLeftPad_StringIntString() {
assertEquals(null, StringUtils.leftPad(null, 5, "-+"));
assertEquals(null, StringUtils.leftPad(null, 5, null));
assertEquals(" ", StringUtils.leftPad("", 5, " "));
assertEquals("-+-+abc", StringUtils.leftPad("abc", 7, "-+"));
assertEquals("-+~abc", StringUtils.leftPad("abc", 6, "-+~"));
assertEquals("-+abc", StringUtils.leftPad("abc", 5, "-+~"));
assertEquals("abc", StringUtils.leftPad("abc", 2, " "));
assertEquals("abc", StringUtils.leftPad("abc", -1, " "));
assertEquals(" abc", StringUtils.leftPad("abc", 5, null));
assertEquals(" abc", StringUtils.leftPad("abc", 5, ""));
}
public void testLength() {
assertEquals(0, StringUtils.length(null));
assertEquals(0, StringUtils.length(""));
assertEquals(0, StringUtils.length(StringUtils.EMPTY));
assertEquals(1, StringUtils.length("A"));
assertEquals(1, StringUtils.length(" "));
assertEquals(8, StringUtils.length("ABCDEFGH"));
}
//-----------------------------------------------------------------------
public void testCenter_StringInt() {
assertEquals(null, StringUtils.center(null, -1));
assertEquals(null, StringUtils.center(null, 4));
assertEquals(" ", StringUtils.center("", 4));
assertEquals("ab", StringUtils.center("ab", 0));
assertEquals("ab", StringUtils.center("ab", -1));
assertEquals("ab", StringUtils.center("ab", 1));
assertEquals(" ", StringUtils.center("", 4));
assertEquals(" ab ", StringUtils.center("ab", 4));
assertEquals("abcd", StringUtils.center("abcd", 2));
assertEquals(" a ", StringUtils.center("a", 4));
assertEquals(" a ", StringUtils.center("a", 5));
}
public void testCenter_StringIntChar() {
assertEquals(null, StringUtils.center(null, -1, ' '));
assertEquals(null, StringUtils.center(null, 4, ' '));
assertEquals(" ", StringUtils.center("", 4, ' '));
assertEquals("ab", StringUtils.center("ab", 0, ' '));
assertEquals("ab", StringUtils.center("ab", -1, ' '));
assertEquals("ab", StringUtils.center("ab", 1, ' '));
assertEquals(" ", StringUtils.center("", 4, ' '));
assertEquals(" ab ", StringUtils.center("ab", 4, ' '));
assertEquals("abcd", StringUtils.center("abcd", 2, ' '));
assertEquals(" a ", StringUtils.center("a", 4, ' '));
assertEquals(" a ", StringUtils.center("a", 5, ' '));
assertEquals("xxaxx", StringUtils.center("a", 5, 'x'));
}
public void testCenter_StringIntString() {
assertEquals(null, StringUtils.center(null, 4, null));
assertEquals(null, StringUtils.center(null, -1, " "));
assertEquals(null, StringUtils.center(null, 4, " "));
assertEquals(" ", StringUtils.center("", 4, " "));
assertEquals("ab", StringUtils.center("ab", 0, " "));
assertEquals("ab", StringUtils.center("ab", -1, " "));
assertEquals("ab", StringUtils.center("ab", 1, " "));
assertEquals(" ", StringUtils.center("", 4, " "));
assertEquals(" ab ", StringUtils.center("ab", 4, " "));
assertEquals("abcd", StringUtils.center("abcd", 2, " "));
assertEquals(" a ", StringUtils.center("a", 4, " "));
assertEquals("yayz", StringUtils.center("a", 4, "yz"));
assertEquals("yzyayzy", StringUtils.center("a", 7, "yz"));
assertEquals(" abc ", StringUtils.center("abc", 7, null));
assertEquals(" abc ", StringUtils.center("abc", 7, ""));
}
//-----------------------------------------------------------------------
public void testReverse_String() {
assertEquals(null, StringUtils.reverse(null) );
assertEquals("", StringUtils.reverse("") );
assertEquals("sdrawkcab", StringUtils.reverse("backwards") );
}
public void testReverseDelimited_StringChar() {
assertEquals(null, StringUtils.reverseDelimited(null, '.') );
assertEquals("", StringUtils.reverseDelimited("", '.') );
assertEquals("c.b.a", StringUtils.reverseDelimited("a.b.c", '.') );
assertEquals("a b c", StringUtils.reverseDelimited("a b c", '.') );
assertEquals("", StringUtils.reverseDelimited("", '.') );
}
//-----------------------------------------------------------------------
public void testDefault_String() {
assertEquals("", StringUtils.defaultString(null));
assertEquals("", StringUtils.defaultString(""));
assertEquals("abc", StringUtils.defaultString("abc"));
}
public void testDefault_StringString() {
assertEquals("NULL", StringUtils.defaultString(null, "NULL"));
assertEquals("", StringUtils.defaultString("", "NULL"));
assertEquals("abc", StringUtils.defaultString("abc", "NULL"));
}
public void testDefaultIfEmpty_StringString() {
assertEquals("NULL", StringUtils.defaultIfEmpty(null, "NULL"));
assertEquals("NULL", StringUtils.defaultIfEmpty("", "NULL"));
assertEquals("abc", StringUtils.defaultIfEmpty("abc", "NULL"));
assertNull(StringUtils.defaultIfEmpty("", null));
}
//-----------------------------------------------------------------------
public void testAbbreviate_StringInt() {
assertEquals(null, StringUtils.abbreviate(null, 10));
assertEquals("", StringUtils.abbreviate("", 10));
assertEquals("short", StringUtils.abbreviate("short", 10));
assertEquals("Now is ...", StringUtils.abbreviate("Now is the time for all good men to come to the aid of their party.", 10));
String raspberry = "raspberry peach";
assertEquals("raspberry p...", StringUtils.abbreviate(raspberry, 14));
assertEquals("raspberry peach", StringUtils.abbreviate("raspberry peach", 15));
assertEquals("raspberry peach", StringUtils.abbreviate("raspberry peach", 16));
assertEquals("abc...", StringUtils.abbreviate("abcdefg", 6));
assertEquals("abcdefg", StringUtils.abbreviate("abcdefg", 7));
assertEquals("abcdefg", StringUtils.abbreviate("abcdefg", 8));
assertEquals("a...", StringUtils.abbreviate("abcdefg", 4));
assertEquals("", StringUtils.abbreviate("", 4));
try {
@SuppressWarnings("unused")
String res = StringUtils.abbreviate("abc", 3);
fail("StringUtils.abbreviate expecting IllegalArgumentException");
} catch (IllegalArgumentException ex) {
// empty
}
}
public void testAbbreviate_StringIntInt() {
assertEquals(null, StringUtils.abbreviate(null, 10, 12));
assertEquals("", StringUtils.abbreviate("", 0, 10));
assertEquals("", StringUtils.abbreviate("", 2, 10));
try {
@SuppressWarnings("unused")
String res = StringUtils.abbreviate("abcdefghij", 0, 3);
fail("StringUtils.abbreviate expecting IllegalArgumentException");
} catch (IllegalArgumentException ex) {
// empty
}
try {
@SuppressWarnings("unused")
String res = StringUtils.abbreviate("abcdefghij", 5, 6);
fail("StringUtils.abbreviate expecting IllegalArgumentException");
} catch (IllegalArgumentException ex) {
// empty
}
String raspberry = "raspberry peach";
assertEquals("raspberry peach", StringUtils.abbreviate(raspberry, 11, 15));
assertEquals(null, StringUtils.abbreviate(null, 7, 14));
assertAbbreviateWithOffset("abcdefg...", -1, 10);
assertAbbreviateWithOffset("abcdefg...", 0, 10);
assertAbbreviateWithOffset("abcdefg...", 1, 10);
assertAbbreviateWithOffset("abcdefg...", 2, 10);
assertAbbreviateWithOffset("abcdefg...", 3, 10);
assertAbbreviateWithOffset("abcdefg...", 4, 10);
assertAbbreviateWithOffset("...fghi...", 5, 10);
assertAbbreviateWithOffset("...ghij...", 6, 10);
assertAbbreviateWithOffset("...hijk...", 7, 10);
assertAbbreviateWithOffset("...ijklmno", 8, 10);
assertAbbreviateWithOffset("...ijklmno", 9, 10);
assertAbbreviateWithOffset("...ijklmno", 10, 10);
assertAbbreviateWithOffset("...ijklmno", 10, 10);
assertAbbreviateWithOffset("...ijklmno", 11, 10);
assertAbbreviateWithOffset("...ijklmno", 12, 10);
assertAbbreviateWithOffset("...ijklmno", 13, 10);
assertAbbreviateWithOffset("...ijklmno", 14, 10);
assertAbbreviateWithOffset("...ijklmno", 15, 10);
assertAbbreviateWithOffset("...ijklmno", 16, 10);
assertAbbreviateWithOffset("...ijklmno", Integer.MAX_VALUE, 10);
}
private void assertAbbreviateWithOffset(String expected, int offset, int maxWidth) {
String abcdefghijklmno = "abcdefghijklmno";
String message = "abbreviate(String,int,int) failed";
String actual = StringUtils.abbreviate(abcdefghijklmno, offset, maxWidth);
if (offset >= 0 && offset < abcdefghijklmno.length()) {
assertTrue(message + " -- should contain offset character",
actual.indexOf((char)('a'+offset)) != -1);
}
assertTrue(message + " -- should not be greater than maxWidth",
actual.length() <= maxWidth);
assertEquals(message, expected, actual);
}
//-----------------------------------------------------------------------
public void testDifference_StringString() {
assertEquals(null, StringUtils.difference(null, null));
assertEquals("", StringUtils.difference("", ""));
assertEquals("abc", StringUtils.difference("", "abc"));
assertEquals("", StringUtils.difference("abc", ""));
assertEquals("i am a robot", StringUtils.difference(null, "i am a robot"));
assertEquals("i am a machine", StringUtils.difference("i am a machine", null));
assertEquals("robot", StringUtils.difference("i am a machine", "i am a robot"));
assertEquals("", StringUtils.difference("abc", "abc"));
assertEquals("you are a robot", StringUtils.difference("i am a robot", "you are a robot"));
}
public void testDifferenceAt_StringString() {
assertEquals(-1, StringUtils.indexOfDifference(null, null));
assertEquals(0, StringUtils.indexOfDifference(null, "i am a robot"));
assertEquals(-1, StringUtils.indexOfDifference("", ""));
assertEquals(0, StringUtils.indexOfDifference("", "abc"));
assertEquals(0, StringUtils.indexOfDifference("abc", ""));
assertEquals(0, StringUtils.indexOfDifference("i am a machine", null));
assertEquals(7, StringUtils.indexOfDifference("i am a machine", "i am a robot"));
assertEquals(-1, StringUtils.indexOfDifference("foo", "foo"));
assertEquals(0, StringUtils.indexOfDifference("i am a robot", "you are a robot"));
//System.out.println("indexOfDiff: " + StringUtils.indexOfDifference("i am a robot", "not machine"));
}
//-----------------------------------------------------------------------
public void testGetLevenshteinDistance_StringString() {
assertEquals(0, StringUtils.getLevenshteinDistance("", "") );
assertEquals(1, StringUtils.getLevenshteinDistance("", "a") );
assertEquals(7, StringUtils.getLevenshteinDistance("aaapppp", "") );
assertEquals(1, StringUtils.getLevenshteinDistance("frog", "fog") );
assertEquals(3, StringUtils.getLevenshteinDistance("fly", "ant") );
assertEquals(7, StringUtils.getLevenshteinDistance("elephant", "hippo") );
assertEquals(7, StringUtils.getLevenshteinDistance("hippo", "elephant") );
assertEquals(8, StringUtils.getLevenshteinDistance("hippo", "zzzzzzzz") );
assertEquals(8, StringUtils.getLevenshteinDistance("zzzzzzzz", "hippo") );
assertEquals(1, StringUtils.getLevenshteinDistance("hello", "hallo") );
try {
@SuppressWarnings("unused")
int d = StringUtils.getLevenshteinDistance("a", null);
fail("expecting IllegalArgumentException");
} catch (IllegalArgumentException ex) {
// empty
}
try {
@SuppressWarnings("unused")
int d = StringUtils.getLevenshteinDistance(null, "a");
fail("expecting IllegalArgumentException");
} catch (IllegalArgumentException ex) {
// empty
}
}
/**
* A sanity check for {@link StringUtils#EMPTY}.
*/
public void testEMPTY() {
assertNotNull(StringUtils.EMPTY);
assertEquals("", StringUtils.EMPTY);
assertEquals(0, StringUtils.EMPTY.length());
}
/**
* Test for {@link StringUtils#isAllLowerCase(String)}.
*/
public void testIsAllLowerCase() {
assertFalse(StringUtils.isAllLowerCase(null));
assertFalse(StringUtils.isAllLowerCase(StringUtils.EMPTY));
assertTrue(StringUtils.isAllLowerCase("abc"));
assertFalse(StringUtils.isAllLowerCase("abc "));
assertFalse(StringUtils.isAllLowerCase("abC"));
}
/**
* Test for {@link StringUtils#isAllUpperCase(String)}.
*/
public void testIsAllUpperCase() {
assertFalse(StringUtils.isAllUpperCase(null));
assertFalse(StringUtils.isAllUpperCase(StringUtils.EMPTY));
assertTrue(StringUtils.isAllUpperCase("ABC"));
assertFalse(StringUtils.isAllUpperCase("ABC "));
assertFalse(StringUtils.isAllUpperCase("aBC"));
}
public void testRemoveStart() {
// StringUtils.removeStart("", *) = ""
assertNull(StringUtils.removeStart(null, null));
assertNull(StringUtils.removeStart(null, ""));
assertNull(StringUtils.removeStart(null, "a"));
// StringUtils.removeStart(*, null) = *
assertEquals(StringUtils.removeStart("", null), "");
assertEquals(StringUtils.removeStart("", ""), "");
assertEquals(StringUtils.removeStart("", "a"), "");
// All others:
assertEquals(StringUtils.removeStart("www.domain.com", "www."), "domain.com");
assertEquals(StringUtils.removeStart("domain.com", "www."), "domain.com");
assertEquals(StringUtils.removeStart("domain.com", ""), "domain.com");
assertEquals(StringUtils.removeStart("domain.com", null), "domain.com");
}
public void testRemoveStartIgnoreCase() {
// StringUtils.removeStart("", *) = ""
assertNull("removeStartIgnoreCase(null, null)", StringUtils.removeStartIgnoreCase(null, null));
assertNull("removeStartIgnoreCase(null, \"\")", StringUtils.removeStartIgnoreCase(null, ""));
assertNull("removeStartIgnoreCase(null, \"a\")", StringUtils.removeStartIgnoreCase(null, "a"));
// StringUtils.removeStart(*, null) = *
assertEquals("removeStartIgnoreCase(\"\", null)", StringUtils.removeStartIgnoreCase("", null), "");
assertEquals("removeStartIgnoreCase(\"\", \"\")", StringUtils.removeStartIgnoreCase("", ""), "");
assertEquals("removeStartIgnoreCase(\"\", \"a\")", StringUtils.removeStartIgnoreCase("", "a"), "");
// All others:
assertEquals("removeStartIgnoreCase(\"www.domain.com\", \"www.\")", StringUtils.removeStartIgnoreCase("www.domain.com", "www."), "domain.com");
assertEquals("removeStartIgnoreCase(\"domain.com\", \"www.\")", StringUtils.removeStartIgnoreCase("domain.com", "www."), "domain.com");
assertEquals("removeStartIgnoreCase(\"domain.com\", \"\")", StringUtils.removeStartIgnoreCase("domain.com", ""), "domain.com");
assertEquals("removeStartIgnoreCase(\"domain.com\", null)", StringUtils.removeStartIgnoreCase("domain.com", null), "domain.com");
// Case insensitive:
assertEquals("removeStartIgnoreCase(\"www.domain.com\", \"WWW.\")", StringUtils.removeStartIgnoreCase("www.domain.com", "WWW."), "domain.com");
}
public void testRemoveEnd() {
// StringUtils.removeEnd("", *) = ""
assertNull(StringUtils.removeEnd(null, null));
assertNull(StringUtils.removeEnd(null, ""));
assertNull(StringUtils.removeEnd(null, "a"));
// StringUtils.removeEnd(*, null) = *
assertEquals(StringUtils.removeEnd("", null), "");
assertEquals(StringUtils.removeEnd("", ""), "");
assertEquals(StringUtils.removeEnd("", "a"), "");
// All others:
assertEquals(StringUtils.removeEnd("www.domain.com.", ".com"), "www.domain.com.");
assertEquals(StringUtils.removeEnd("www.domain.com", ".com"), "www.domain");
assertEquals(StringUtils.removeEnd("www.domain", ".com"), "www.domain");
assertEquals(StringUtils.removeEnd("domain.com", ""), "domain.com");
assertEquals(StringUtils.removeEnd("domain.com", null), "domain.com");
}
public void testRemoveEndIgnoreCase() {
// StringUtils.removeEndIgnoreCase("", *) = ""
assertNull("removeEndIgnoreCase(null, null)", StringUtils.removeEndIgnoreCase(null, null));
assertNull("removeEndIgnoreCase(null, \"\")", StringUtils.removeEndIgnoreCase(null, ""));
assertNull("removeEndIgnoreCase(null, \"a\")", StringUtils.removeEndIgnoreCase(null, "a"));
// StringUtils.removeEnd(*, null) = *
assertEquals("removeEndIgnoreCase(\"\", null)", StringUtils.removeEndIgnoreCase("", null), "");
assertEquals("removeEndIgnoreCase(\"\", \"\")", StringUtils.removeEndIgnoreCase("", ""), "");
assertEquals("removeEndIgnoreCase(\"\", \"a\")", StringUtils.removeEndIgnoreCase("", "a"), "");
// All others:
assertEquals("removeEndIgnoreCase(\"www.domain.com.\", \".com\")", StringUtils.removeEndIgnoreCase("www.domain.com.", ".com"), "www.domain.com.");
assertEquals("removeEndIgnoreCase(\"www.domain.com\", \".com\")", StringUtils.removeEndIgnoreCase("www.domain.com", ".com"), "www.domain");
assertEquals("removeEndIgnoreCase(\"www.domain\", \".com\")", StringUtils.removeEndIgnoreCase("www.domain", ".com"), "www.domain");
assertEquals("removeEndIgnoreCase(\"domain.com\", \"\")", StringUtils.removeEndIgnoreCase("domain.com", ""), "domain.com");
assertEquals("removeEndIgnoreCase(\"domain.com\", null)", StringUtils.removeEndIgnoreCase("domain.com", null), "domain.com");
// Case insensitive:
assertEquals("removeEndIgnoreCase(\"www.domain.com\", \".com\")", StringUtils.removeEndIgnoreCase("www.domain.com", ".COM"), "www.domain");
}
public void testRemove_String() {
// StringUtils.remove(null, *) = null
assertEquals(null, StringUtils.remove(null, null));
assertEquals(null, StringUtils.remove(null, ""));
assertEquals(null, StringUtils.remove(null, "a"));
// StringUtils.remove("", *) = ""
assertEquals("", StringUtils.remove("", null));
assertEquals("", StringUtils.remove("", ""));
assertEquals("", StringUtils.remove("", "a"));
// StringUtils.remove(*, null) = *
assertEquals(null, StringUtils.remove(null, null));
assertEquals("", StringUtils.remove("", null));
assertEquals("a", StringUtils.remove("a", null));
// StringUtils.remove(*, "") = *
assertEquals(null, StringUtils.remove(null, ""));
assertEquals("", StringUtils.remove("", ""));
assertEquals("a", StringUtils.remove("a", ""));
// StringUtils.remove("queued", "ue") = "qd"
assertEquals("qd", StringUtils.remove("queued", "ue"));
// StringUtils.remove("queued", "zz") = "queued"
assertEquals("queued", StringUtils.remove("queued", "zz"));
}
public void testRemove_char() {
// StringUtils.remove(null, *) = null
assertEquals(null, StringUtils.remove(null, 'a'));
assertEquals(null, StringUtils.remove(null, 'a'));
assertEquals(null, StringUtils.remove(null, 'a'));
// StringUtils.remove("", *) = ""
assertEquals("", StringUtils.remove("", 'a'));
assertEquals("", StringUtils.remove("", 'a'));
assertEquals("", StringUtils.remove("", 'a'));
// StringUtils.remove("queued", 'u') = "qeed"
assertEquals("qeed", StringUtils.remove("queued", 'u'));
// StringUtils.remove("queued", 'z') = "queued"
assertEquals("queued", StringUtils.remove("queued", 'z'));
}
public void testDifferenceAt_StringArray(){
assertEquals(-1, StringUtils.indexOfDifference(null));
assertEquals(-1, StringUtils.indexOfDifference(new String[] {}));
assertEquals(-1, StringUtils.indexOfDifference(new String[] {"abc"}));
assertEquals(-1, StringUtils.indexOfDifference(new String[] {null, null}));
assertEquals(-1, StringUtils.indexOfDifference(new String[] {"", ""}));
assertEquals(0, StringUtils.indexOfDifference(new String[] {"", null}));
assertEquals(0, StringUtils.indexOfDifference(new String[] {"abc", null, null}));
assertEquals(0, StringUtils.indexOfDifference(new String[] {null, null, "abc"}));
assertEquals(0, StringUtils.indexOfDifference(new String[] {"", "abc"}));
assertEquals(0, StringUtils.indexOfDifference(new String[] {"abc", ""}));
assertEquals(-1, StringUtils.indexOfDifference(new String[] {"abc", "abc"}));
assertEquals(1, StringUtils.indexOfDifference(new String[] {"abc", "a"}));
assertEquals(2, StringUtils.indexOfDifference(new String[] {"ab", "abxyz"}));
assertEquals(2, StringUtils.indexOfDifference(new String[] {"abcde", "abxyz"}));
assertEquals(0, StringUtils.indexOfDifference(new String[] {"abcde", "xyz"}));
assertEquals(0, StringUtils.indexOfDifference(new String[] {"xyz", "abcde"}));
assertEquals(7, StringUtils.indexOfDifference(new String[] {"i am a machine", "i am a robot"}));
}
public void testGetCommonPrefix_StringArray(){
assertEquals("", StringUtils.getCommonPrefix(null));
assertEquals("", StringUtils.getCommonPrefix(new String[] {}));
assertEquals("abc", StringUtils.getCommonPrefix(new String[] {"abc"}));
assertEquals("", StringUtils.getCommonPrefix(new String[] {null, null}));
assertEquals("", StringUtils.getCommonPrefix(new String[] {"", ""}));
assertEquals("", StringUtils.getCommonPrefix(new String[] {"", null}));
assertEquals("", StringUtils.getCommonPrefix(new String[] {"abc", null, null}));
assertEquals("", StringUtils.getCommonPrefix(new String[] {null, null, "abc"}));
assertEquals("", StringUtils.getCommonPrefix(new String[] {"", "abc"}));
assertEquals("", StringUtils.getCommonPrefix(new String[] {"abc", ""}));
assertEquals("abc", StringUtils.getCommonPrefix(new String[] {"abc", "abc"}));
assertEquals("a", StringUtils.getCommonPrefix(new String[] {"abc", "a"}));
assertEquals("ab", StringUtils.getCommonPrefix(new String[] {"ab", "abxyz"}));
assertEquals("ab", StringUtils.getCommonPrefix(new String[] {"abcde", "abxyz"}));
assertEquals("", StringUtils.getCommonPrefix(new String[] {"abcde", "xyz"}));
assertEquals("", StringUtils.getCommonPrefix(new String[] {"xyz", "abcde"}));
assertEquals("i am a ", StringUtils.getCommonPrefix(new String[] {"i am a machine", "i am a robot"}));
}
public void testStartsWithAny() {
assertFalse(StringUtils.startsWithAny(null, null));
assertFalse(StringUtils.startsWithAny(null, new String[] {"abc"}));
assertFalse(StringUtils.startsWithAny("abcxyz", null));
assertFalse(StringUtils.startsWithAny("abcxyz", new String[] {}));
assertTrue(StringUtils.startsWithAny("abcxyz", new String[] {"abc"}));
assertTrue(StringUtils.startsWithAny("abcxyz", new String[] {null, "xyz", "abc"}));
assertFalse(StringUtils.startsWithAny("abcxyz", new String[] {null, "xyz", "abcd"}));
}
} |
|
public void testStop3() throws Exception
{
String[] args = new String[]{"--zop==1",
"-abtoast",
"--b=bar"};
CommandLine cl = parser.parse(options, args, true);
assertFalse("Confirm -a is not set", cl.hasOption("a"));
assertFalse("Confirm -b is not set", cl.hasOption("b"));
assertTrue("Confirm 3 extra args: " + cl.getArgList().size(), cl.getArgList().size() == 3);
} | org.apache.commons.cli.PosixParserTest::testStop3 | src/test/org/apache/commons/cli/PosixParserTest.java | 169 | src/test/org/apache/commons/cli/PosixParserTest.java | testStop3 | /**
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.cli;
import java.util.Arrays;
import java.util.List;
import junit.framework.TestCase;
/**
* Test case for the PosixParser.
*
* @version $Revision$, $Date$
*/
public class PosixParserTest extends TestCase
{
private Options options = null;
private Parser parser = null;
public void setUp()
{
options = new Options()
.addOption("a", "enable-a", false, "turn [a] on or off")
.addOption("b", "bfile", true, "set the value of [b]")
.addOption("c", "copt", false, "turn [c] on or off");
parser = new PosixParser();
}
public void testSimpleShort() throws Exception
{
String[] args = new String[] { "-a",
"-b", "toast",
"foo", "bar" };
CommandLine cl = parser.parse(options, args);
assertTrue( "Confirm -a is set", cl.hasOption("a") );
assertTrue( "Confirm -b is set", cl.hasOption("b") );
assertTrue( "Confirm arg of -b", cl.getOptionValue("b").equals("toast") );
assertTrue( "Confirm size of extra args", cl.getArgList().size() == 2);
}
public void testSimpleLong() throws Exception
{
String[] args = new String[] { "--enable-a",
"--bfile", "toast",
"foo", "bar" };
CommandLine cl = parser.parse(options, args);
assertTrue( "Confirm -a is set", cl.hasOption("a") );
assertTrue( "Confirm -b is set", cl.hasOption("b") );
assertTrue( "Confirm arg of -b", cl.getOptionValue("b").equals("toast") );
assertTrue( "Confirm arg of --bfile", cl.getOptionValue( "bfile" ).equals( "toast" ) );
assertTrue( "Confirm size of extra args", cl.getArgList().size() == 2);
}
public void testComplexShort() throws Exception
{
String[] args = new String[] { "-acbtoast",
"foo", "bar" };
CommandLine cl = parser.parse(options, args);
assertTrue( "Confirm -a is set", cl.hasOption("a") );
assertTrue( "Confirm -b is set", cl.hasOption("b") );
assertTrue( "Confirm -c is set", cl.hasOption("c") );
assertTrue( "Confirm arg of -b", cl.getOptionValue("b").equals("toast") );
assertTrue( "Confirm size of extra args", cl.getArgList().size() == 2);
}
public void testUnrecognizedOption() throws Exception
{
String[] args = new String[] { "-adbtoast", "foo", "bar" };
try
{
parser.parse(options, args);
fail("UnrecognizedOptionException wasn't thrown");
}
catch (UnrecognizedOptionException e)
{
assertEquals("-adbtoast", e.getOption());
}
}
public void testUnrecognizedOption2() throws Exception
{
String[] args = new String[] { "-z", "-abtoast", "foo", "bar" };
try
{
parser.parse(options, args);
fail("UnrecognizedOptionException wasn't thrown");
}
catch (UnrecognizedOptionException e)
{
assertEquals("-z", e.getOption());
}
}
public void testMissingArg() throws Exception
{
String[] args = new String[] { "-acb" };
boolean caught = false;
try
{
parser.parse(options, args);
}
catch (MissingArgumentException e)
{
caught = true;
assertEquals("option missing an argument", "b", e.getOption().getOpt());
}
assertTrue( "Confirm MissingArgumentException caught", caught );
}
public void testStop() throws Exception
{
String[] args = new String[] { "-c",
"foober",
"-btoast" };
CommandLine cl = parser.parse(options, args, true);
assertTrue( "Confirm -c is set", cl.hasOption("c") );
assertTrue( "Confirm 2 extra args: " + cl.getArgList().size(), cl.getArgList().size() == 2);
}
public void testStop2() throws Exception
{
String[] args = new String[]{"-z",
"-a",
"-btoast"};
CommandLine cl = parser.parse(options, args, true);
assertFalse("Confirm -a is not set", cl.hasOption("a"));
assertTrue("Confirm 3 extra args: " + cl.getArgList().size(), cl.getArgList().size() == 3);
}
public void testStop3() throws Exception
{
String[] args = new String[]{"--zop==1",
"-abtoast",
"--b=bar"};
CommandLine cl = parser.parse(options, args, true);
assertFalse("Confirm -a is not set", cl.hasOption("a"));
assertFalse("Confirm -b is not set", cl.hasOption("b"));
assertTrue("Confirm 3 extra args: " + cl.getArgList().size(), cl.getArgList().size() == 3);
}
public void testStopBursting() throws Exception
{
String[] args = new String[] { "-azc" };
CommandLine cl = parser.parse(options, args, true);
assertTrue( "Confirm -a is set", cl.hasOption("a") );
assertFalse( "Confirm -c is not set", cl.hasOption("c") );
assertTrue( "Confirm 1 extra arg: " + cl.getArgList().size(), cl.getArgList().size() == 1);
assertTrue(cl.getArgList().contains("zc"));
}
public void testMultiple() throws Exception
{
String[] args = new String[] { "-c",
"foobar",
"-btoast" };
CommandLine cl = parser.parse(options, args, true);
assertTrue( "Confirm -c is set", cl.hasOption("c") );
assertTrue( "Confirm 2 extra args: " + cl.getArgList().size(), cl.getArgList().size() == 2);
cl = parser.parse(options, cl.getArgs() );
assertTrue( "Confirm -c is not set", ! cl.hasOption("c") );
assertTrue( "Confirm -b is set", cl.hasOption("b") );
assertTrue( "Confirm arg of -b", cl.getOptionValue("b").equals("toast") );
assertTrue( "Confirm 1 extra arg: " + cl.getArgList().size(), cl.getArgList().size() == 1);
assertTrue( "Confirm value of extra arg: " + cl.getArgList().get(0), cl.getArgList().get(0).equals("foobar") );
}
public void testMultipleWithLong() throws Exception
{
String[] args = new String[] { "--copt",
"foobar",
"--bfile", "toast" };
CommandLine cl = parser.parse(options,args, true);
assertTrue( "Confirm -c is set", cl.hasOption("c") );
assertTrue( "Confirm 3 extra args: " + cl.getArgList().size(), cl.getArgList().size() == 3);
cl = parser.parse(options, cl.getArgs() );
assertTrue( "Confirm -c is not set", ! cl.hasOption("c") );
assertTrue( "Confirm -b is set", cl.hasOption("b") );
assertTrue( "Confirm arg of -b", cl.getOptionValue("b").equals("toast") );
assertTrue( "Confirm 1 extra arg: " + cl.getArgList().size(), cl.getArgList().size() == 1);
assertTrue( "Confirm value of extra arg: " + cl.getArgList().get(0), cl.getArgList().get(0).equals("foobar") );
}
public void testDoubleDash() throws Exception
{
String[] args = new String[] { "--copt",
"--",
"-b", "toast" };
CommandLine cl = parser.parse(options, args);
assertTrue( "Confirm -c is set", cl.hasOption("c") );
assertTrue( "Confirm -b is not set", ! cl.hasOption("b") );
assertTrue( "Confirm 2 extra args: " + cl.getArgList().size(), cl.getArgList().size() == 2);
}
public void testSingleDash() throws Exception
{
String[] args = new String[] { "--copt",
"-b", "-",
"-a",
"-" };
CommandLine cl = parser.parse(options, args);
assertTrue( "Confirm -a is set", cl.hasOption("a") );
assertTrue( "Confirm -b is set", cl.hasOption("b") );
assertTrue( "Confirm arg of -b", cl.getOptionValue("b").equals("-") );
assertTrue( "Confirm 1 extra arg: " + cl.getArgList().size(), cl.getArgList().size() == 1);
assertTrue( "Confirm value of extra arg: " + cl.getArgList().get(0), cl.getArgList().get(0).equals("-") );
}
/**
* Real world test with long and short options.
*/
public void testLongOptionWithShort() throws Exception {
Option help = new Option("h", "help", false, "print this message");
Option version = new Option("v", "version", false, "print version information");
Option newRun = new Option("n", "new", false, "Create NLT cache entries only for new items");
Option trackerRun = new Option("t", "tracker", false, "Create NLT cache entries only for tracker items");
Option timeLimit = OptionBuilder.withLongOpt("limit").hasArg()
.withValueSeparator()
.withDescription("Set time limit for execution, in mintues")
.create("l");
Option age = OptionBuilder.withLongOpt("age").hasArg()
.withValueSeparator()
.withDescription("Age (in days) of cache item before being recomputed")
.create("a");
Option server = OptionBuilder.withLongOpt("server").hasArg()
.withValueSeparator()
.withDescription("The NLT server address")
.create("s");
Option numResults = OptionBuilder.withLongOpt("results").hasArg()
.withValueSeparator()
.withDescription("Number of results per item")
.create("r");
Option configFile = OptionBuilder.withLongOpt("file").hasArg()
.withValueSeparator()
.withDescription("Use the specified configuration file")
.create();
Options options = new Options();
options.addOption(help);
options.addOption(version);
options.addOption(newRun);
options.addOption(trackerRun);
options.addOption(timeLimit);
options.addOption(age);
options.addOption(server);
options.addOption(numResults);
options.addOption(configFile);
// create the command line parser
CommandLineParser parser = new PosixParser();
String[] args = new String[] {
"-v",
"-l",
"10",
"-age",
"5",
"-file",
"filename"
};
CommandLine line = parser.parse(options, args);
assertTrue(line.hasOption("v"));
assertEquals(line.getOptionValue("l"), "10");
assertEquals(line.getOptionValue("limit"), "10");
assertEquals(line.getOptionValue("a"), "5");
assertEquals(line.getOptionValue("age"), "5");
assertEquals(line.getOptionValue("file"), "filename");
}
public void testPropertiesOption() throws Exception
{
String[] args = new String[] { "-Jsource=1.5", "-J", "target", "1.5", "foo" };
Options options = new Options();
options.addOption(OptionBuilder.withValueSeparator().hasArgs(2).create('J'));
Parser parser = new PosixParser();
CommandLine cl = parser.parse(options, args);
List values = Arrays.asList(cl.getOptionValues("J"));
assertNotNull("null values", values);
assertEquals("number of values", 4, values.size());
assertEquals("value 1", "source", values.get(0));
assertEquals("value 2", "1.5", values.get(1));
assertEquals("value 3", "target", values.get(2));
assertEquals("value 4", "1.5", values.get(3));
List argsleft = cl.getArgList();
assertEquals("Should be 1 arg left",1,argsleft.size());
assertEquals("Expecting foo","foo",argsleft.get(0));
}
} | // You are a professional Java test case writer, please create a test case named `testStop3` for the issue `Cli-CLI-165`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Cli-CLI-165
//
// ## Issue-Title:
// PosixParser keeps processing tokens after a non unrecognized long option
//
// ## Issue-Description:
//
// PosixParser keeps processing tokens after a non unrecognized long option when stopAtNonOption is enabled. The tokens after the unrecognized long option are burst, split around '=', etc.. instead of being kept as is.
//
//
// For example, with the options 'a' and 'b' defined, 'b' having an argument, the following command line:
//
//
//
//
// ```
// --zop -abfoo
// ```
//
//
// is interpreted as:
//
//
//
//
// ```
// --zop -a -b foo
// ```
//
//
// but the last token should remain unchanged.
//
//
//
//
//
public void testStop3() throws Exception {
| 169 | 20 | 158 | src/test/org/apache/commons/cli/PosixParserTest.java | src/test | ```markdown
## Issue-ID: Cli-CLI-165
## Issue-Title:
PosixParser keeps processing tokens after a non unrecognized long option
## Issue-Description:
PosixParser keeps processing tokens after a non unrecognized long option when stopAtNonOption is enabled. The tokens after the unrecognized long option are burst, split around '=', etc.. instead of being kept as is.
For example, with the options 'a' and 'b' defined, 'b' having an argument, the following command line:
```
--zop -abfoo
```
is interpreted as:
```
--zop -a -b foo
```
but the last token should remain unchanged.
```
You are a professional Java test case writer, please create a test case named `testStop3` for the issue `Cli-CLI-165`, utilizing the provided issue report information and the following function signature.
```java
public void testStop3() throws Exception {
```
| 158 | [
"org.apache.commons.cli.PosixParser"
] | 90ce3e7767e044abbbedd69e07cc95a54688b9bf52d0f9be0567f416e6ae44e4 | public void testStop3() throws Exception
| // You are a professional Java test case writer, please create a test case named `testStop3` for the issue `Cli-CLI-165`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Cli-CLI-165
//
// ## Issue-Title:
// PosixParser keeps processing tokens after a non unrecognized long option
//
// ## Issue-Description:
//
// PosixParser keeps processing tokens after a non unrecognized long option when stopAtNonOption is enabled. The tokens after the unrecognized long option are burst, split around '=', etc.. instead of being kept as is.
//
//
// For example, with the options 'a' and 'b' defined, 'b' having an argument, the following command line:
//
//
//
//
// ```
// --zop -abfoo
// ```
//
//
// is interpreted as:
//
//
//
//
// ```
// --zop -a -b foo
// ```
//
//
// but the last token should remain unchanged.
//
//
//
//
//
| Cli | /**
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.cli;
import java.util.Arrays;
import java.util.List;
import junit.framework.TestCase;
/**
* Test case for the PosixParser.
*
* @version $Revision$, $Date$
*/
public class PosixParserTest extends TestCase
{
private Options options = null;
private Parser parser = null;
public void setUp()
{
options = new Options()
.addOption("a", "enable-a", false, "turn [a] on or off")
.addOption("b", "bfile", true, "set the value of [b]")
.addOption("c", "copt", false, "turn [c] on or off");
parser = new PosixParser();
}
public void testSimpleShort() throws Exception
{
String[] args = new String[] { "-a",
"-b", "toast",
"foo", "bar" };
CommandLine cl = parser.parse(options, args);
assertTrue( "Confirm -a is set", cl.hasOption("a") );
assertTrue( "Confirm -b is set", cl.hasOption("b") );
assertTrue( "Confirm arg of -b", cl.getOptionValue("b").equals("toast") );
assertTrue( "Confirm size of extra args", cl.getArgList().size() == 2);
}
public void testSimpleLong() throws Exception
{
String[] args = new String[] { "--enable-a",
"--bfile", "toast",
"foo", "bar" };
CommandLine cl = parser.parse(options, args);
assertTrue( "Confirm -a is set", cl.hasOption("a") );
assertTrue( "Confirm -b is set", cl.hasOption("b") );
assertTrue( "Confirm arg of -b", cl.getOptionValue("b").equals("toast") );
assertTrue( "Confirm arg of --bfile", cl.getOptionValue( "bfile" ).equals( "toast" ) );
assertTrue( "Confirm size of extra args", cl.getArgList().size() == 2);
}
public void testComplexShort() throws Exception
{
String[] args = new String[] { "-acbtoast",
"foo", "bar" };
CommandLine cl = parser.parse(options, args);
assertTrue( "Confirm -a is set", cl.hasOption("a") );
assertTrue( "Confirm -b is set", cl.hasOption("b") );
assertTrue( "Confirm -c is set", cl.hasOption("c") );
assertTrue( "Confirm arg of -b", cl.getOptionValue("b").equals("toast") );
assertTrue( "Confirm size of extra args", cl.getArgList().size() == 2);
}
public void testUnrecognizedOption() throws Exception
{
String[] args = new String[] { "-adbtoast", "foo", "bar" };
try
{
parser.parse(options, args);
fail("UnrecognizedOptionException wasn't thrown");
}
catch (UnrecognizedOptionException e)
{
assertEquals("-adbtoast", e.getOption());
}
}
public void testUnrecognizedOption2() throws Exception
{
String[] args = new String[] { "-z", "-abtoast", "foo", "bar" };
try
{
parser.parse(options, args);
fail("UnrecognizedOptionException wasn't thrown");
}
catch (UnrecognizedOptionException e)
{
assertEquals("-z", e.getOption());
}
}
public void testMissingArg() throws Exception
{
String[] args = new String[] { "-acb" };
boolean caught = false;
try
{
parser.parse(options, args);
}
catch (MissingArgumentException e)
{
caught = true;
assertEquals("option missing an argument", "b", e.getOption().getOpt());
}
assertTrue( "Confirm MissingArgumentException caught", caught );
}
public void testStop() throws Exception
{
String[] args = new String[] { "-c",
"foober",
"-btoast" };
CommandLine cl = parser.parse(options, args, true);
assertTrue( "Confirm -c is set", cl.hasOption("c") );
assertTrue( "Confirm 2 extra args: " + cl.getArgList().size(), cl.getArgList().size() == 2);
}
public void testStop2() throws Exception
{
String[] args = new String[]{"-z",
"-a",
"-btoast"};
CommandLine cl = parser.parse(options, args, true);
assertFalse("Confirm -a is not set", cl.hasOption("a"));
assertTrue("Confirm 3 extra args: " + cl.getArgList().size(), cl.getArgList().size() == 3);
}
public void testStop3() throws Exception
{
String[] args = new String[]{"--zop==1",
"-abtoast",
"--b=bar"};
CommandLine cl = parser.parse(options, args, true);
assertFalse("Confirm -a is not set", cl.hasOption("a"));
assertFalse("Confirm -b is not set", cl.hasOption("b"));
assertTrue("Confirm 3 extra args: " + cl.getArgList().size(), cl.getArgList().size() == 3);
}
public void testStopBursting() throws Exception
{
String[] args = new String[] { "-azc" };
CommandLine cl = parser.parse(options, args, true);
assertTrue( "Confirm -a is set", cl.hasOption("a") );
assertFalse( "Confirm -c is not set", cl.hasOption("c") );
assertTrue( "Confirm 1 extra arg: " + cl.getArgList().size(), cl.getArgList().size() == 1);
assertTrue(cl.getArgList().contains("zc"));
}
public void testMultiple() throws Exception
{
String[] args = new String[] { "-c",
"foobar",
"-btoast" };
CommandLine cl = parser.parse(options, args, true);
assertTrue( "Confirm -c is set", cl.hasOption("c") );
assertTrue( "Confirm 2 extra args: " + cl.getArgList().size(), cl.getArgList().size() == 2);
cl = parser.parse(options, cl.getArgs() );
assertTrue( "Confirm -c is not set", ! cl.hasOption("c") );
assertTrue( "Confirm -b is set", cl.hasOption("b") );
assertTrue( "Confirm arg of -b", cl.getOptionValue("b").equals("toast") );
assertTrue( "Confirm 1 extra arg: " + cl.getArgList().size(), cl.getArgList().size() == 1);
assertTrue( "Confirm value of extra arg: " + cl.getArgList().get(0), cl.getArgList().get(0).equals("foobar") );
}
public void testMultipleWithLong() throws Exception
{
String[] args = new String[] { "--copt",
"foobar",
"--bfile", "toast" };
CommandLine cl = parser.parse(options,args, true);
assertTrue( "Confirm -c is set", cl.hasOption("c") );
assertTrue( "Confirm 3 extra args: " + cl.getArgList().size(), cl.getArgList().size() == 3);
cl = parser.parse(options, cl.getArgs() );
assertTrue( "Confirm -c is not set", ! cl.hasOption("c") );
assertTrue( "Confirm -b is set", cl.hasOption("b") );
assertTrue( "Confirm arg of -b", cl.getOptionValue("b").equals("toast") );
assertTrue( "Confirm 1 extra arg: " + cl.getArgList().size(), cl.getArgList().size() == 1);
assertTrue( "Confirm value of extra arg: " + cl.getArgList().get(0), cl.getArgList().get(0).equals("foobar") );
}
public void testDoubleDash() throws Exception
{
String[] args = new String[] { "--copt",
"--",
"-b", "toast" };
CommandLine cl = parser.parse(options, args);
assertTrue( "Confirm -c is set", cl.hasOption("c") );
assertTrue( "Confirm -b is not set", ! cl.hasOption("b") );
assertTrue( "Confirm 2 extra args: " + cl.getArgList().size(), cl.getArgList().size() == 2);
}
public void testSingleDash() throws Exception
{
String[] args = new String[] { "--copt",
"-b", "-",
"-a",
"-" };
CommandLine cl = parser.parse(options, args);
assertTrue( "Confirm -a is set", cl.hasOption("a") );
assertTrue( "Confirm -b is set", cl.hasOption("b") );
assertTrue( "Confirm arg of -b", cl.getOptionValue("b").equals("-") );
assertTrue( "Confirm 1 extra arg: " + cl.getArgList().size(), cl.getArgList().size() == 1);
assertTrue( "Confirm value of extra arg: " + cl.getArgList().get(0), cl.getArgList().get(0).equals("-") );
}
/**
* Real world test with long and short options.
*/
public void testLongOptionWithShort() throws Exception {
Option help = new Option("h", "help", false, "print this message");
Option version = new Option("v", "version", false, "print version information");
Option newRun = new Option("n", "new", false, "Create NLT cache entries only for new items");
Option trackerRun = new Option("t", "tracker", false, "Create NLT cache entries only for tracker items");
Option timeLimit = OptionBuilder.withLongOpt("limit").hasArg()
.withValueSeparator()
.withDescription("Set time limit for execution, in mintues")
.create("l");
Option age = OptionBuilder.withLongOpt("age").hasArg()
.withValueSeparator()
.withDescription("Age (in days) of cache item before being recomputed")
.create("a");
Option server = OptionBuilder.withLongOpt("server").hasArg()
.withValueSeparator()
.withDescription("The NLT server address")
.create("s");
Option numResults = OptionBuilder.withLongOpt("results").hasArg()
.withValueSeparator()
.withDescription("Number of results per item")
.create("r");
Option configFile = OptionBuilder.withLongOpt("file").hasArg()
.withValueSeparator()
.withDescription("Use the specified configuration file")
.create();
Options options = new Options();
options.addOption(help);
options.addOption(version);
options.addOption(newRun);
options.addOption(trackerRun);
options.addOption(timeLimit);
options.addOption(age);
options.addOption(server);
options.addOption(numResults);
options.addOption(configFile);
// create the command line parser
CommandLineParser parser = new PosixParser();
String[] args = new String[] {
"-v",
"-l",
"10",
"-age",
"5",
"-file",
"filename"
};
CommandLine line = parser.parse(options, args);
assertTrue(line.hasOption("v"));
assertEquals(line.getOptionValue("l"), "10");
assertEquals(line.getOptionValue("limit"), "10");
assertEquals(line.getOptionValue("a"), "5");
assertEquals(line.getOptionValue("age"), "5");
assertEquals(line.getOptionValue("file"), "filename");
}
public void testPropertiesOption() throws Exception
{
String[] args = new String[] { "-Jsource=1.5", "-J", "target", "1.5", "foo" };
Options options = new Options();
options.addOption(OptionBuilder.withValueSeparator().hasArgs(2).create('J'));
Parser parser = new PosixParser();
CommandLine cl = parser.parse(options, args);
List values = Arrays.asList(cl.getOptionValues("J"));
assertNotNull("null values", values);
assertEquals("number of values", 4, values.size());
assertEquals("value 1", "source", values.get(0));
assertEquals("value 2", "1.5", values.get(1));
assertEquals("value 3", "target", values.get(2));
assertEquals("value 4", "1.5", values.get(3));
List argsleft = cl.getArgList();
assertEquals("Should be 1 arg left",1,argsleft.size());
assertEquals("Expecting foo","foo",argsleft.get(0));
}
} |
||
@Test
public void testTooLargeFirstStep() {
AdaptiveStepsizeIntegrator integ =
new DormandPrince853Integrator(0, Double.POSITIVE_INFINITY, Double.NaN, Double.NaN);
final double start = 0.0;
final double end = 0.001;
FirstOrderDifferentialEquations equations = new FirstOrderDifferentialEquations() {
public int getDimension() {
return 1;
}
public void computeDerivatives(double t, double[] y, double[] yDot) {
Assert.assertTrue(t >= FastMath.nextAfter(start, Double.NEGATIVE_INFINITY));
Assert.assertTrue(t <= FastMath.nextAfter(end, Double.POSITIVE_INFINITY));
yDot[0] = -100.0 * y[0];
}
};
integ.setStepSizeControl(0, 1.0, 1.0e-6, 1.0e-8);
integ.integrate(equations, start, new double[] { 1.0 }, end, new double[1]);
} | org.apache.commons.math.ode.nonstiff.DormandPrince853IntegratorTest::testTooLargeFirstStep | src/test/java/org/apache/commons/math/ode/nonstiff/DormandPrince853IntegratorTest.java | 202 | src/test/java/org/apache/commons/math/ode/nonstiff/DormandPrince853IntegratorTest.java | testTooLargeFirstStep | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.math.ode.nonstiff;
import org.apache.commons.math.exception.DimensionMismatchException;
import org.apache.commons.math.exception.NumberIsTooSmallException;
import org.apache.commons.math.ode.FirstOrderDifferentialEquations;
import org.apache.commons.math.ode.FirstOrderIntegrator;
import org.apache.commons.math.ode.TestProblem1;
import org.apache.commons.math.ode.TestProblem3;
import org.apache.commons.math.ode.TestProblem4;
import org.apache.commons.math.ode.TestProblem5;
import org.apache.commons.math.ode.TestProblemHandler;
import org.apache.commons.math.ode.events.EventHandler;
import org.apache.commons.math.ode.sampling.StepHandler;
import org.apache.commons.math.ode.sampling.StepInterpolator;
import org.apache.commons.math.util.FastMath;
import org.junit.Assert;
import org.junit.Test;
public class DormandPrince853IntegratorTest {
@Test
public void testMissedEndEvent() {
final double t0 = 1878250320.0000029;
final double tEvent = 1878250379.9999986;
final double[] k = { 1.0e-4, 1.0e-5, 1.0e-6 };
FirstOrderDifferentialEquations ode = new FirstOrderDifferentialEquations() {
public int getDimension() {
return k.length;
}
public void computeDerivatives(double t, double[] y, double[] yDot) {
for (int i = 0; i < y.length; ++i) {
yDot[i] = k[i] * y[i];
}
}
};
DormandPrince853Integrator integrator = new DormandPrince853Integrator(0.0, 100.0,
1.0e-10, 1.0e-10);
double[] y0 = new double[k.length];
for (int i = 0; i < y0.length; ++i) {
y0[i] = i + 1;
}
double[] y = new double[k.length];
integrator.setInitialStepSize(60.0);
double finalT = integrator.integrate(ode, t0, y0, tEvent, y);
Assert.assertEquals(tEvent, finalT, 5.0e-6);
for (int i = 0; i < y.length; ++i) {
Assert.assertEquals(y0[i] * FastMath.exp(k[i] * (finalT - t0)), y[i], 1.0e-9);
}
integrator.setInitialStepSize(60.0);
integrator.addEventHandler(new EventHandler() {
public void init(double t0, double[] y0, double t) {
}
public void resetState(double t, double[] y) {
}
public double g(double t, double[] y) {
return t - tEvent;
}
public Action eventOccurred(double t, double[] y, boolean increasing) {
Assert.assertEquals(tEvent, t, 5.0e-6);
return Action.CONTINUE;
}
}, Double.POSITIVE_INFINITY, 1.0e-20, 100);
finalT = integrator.integrate(ode, t0, y0, tEvent + 120, y);
Assert.assertEquals(tEvent + 120, finalT, 5.0e-6);
for (int i = 0; i < y.length; ++i) {
Assert.assertEquals(y0[i] * FastMath.exp(k[i] * (finalT - t0)), y[i], 1.0e-9);
}
}
@Test(expected=DimensionMismatchException.class)
public void testDimensionCheck() {
TestProblem1 pb = new TestProblem1();
DormandPrince853Integrator integrator = new DormandPrince853Integrator(0.0, 1.0,
1.0e-10, 1.0e-10);
integrator.integrate(pb,
0.0, new double[pb.getDimension()+10],
1.0, new double[pb.getDimension()+10]);
Assert.fail("an exception should have been thrown");
}
@Test(expected=NumberIsTooSmallException.class)
public void testNullIntervalCheck() {
TestProblem1 pb = new TestProblem1();
DormandPrince853Integrator integrator = new DormandPrince853Integrator(0.0, 1.0,
1.0e-10, 1.0e-10);
integrator.integrate(pb,
0.0, new double[pb.getDimension()],
0.0, new double[pb.getDimension()]);
Assert.fail("an exception should have been thrown");
}
@Test(expected=NumberIsTooSmallException.class)
public void testMinStep() {
TestProblem1 pb = new TestProblem1();
double minStep = 0.1 * (pb.getFinalTime() - pb.getInitialTime());
double maxStep = pb.getFinalTime() - pb.getInitialTime();
double[] vecAbsoluteTolerance = { 1.0e-15, 1.0e-16 };
double[] vecRelativeTolerance = { 1.0e-15, 1.0e-16 };
FirstOrderIntegrator integ = new DormandPrince853Integrator(minStep, maxStep,
vecAbsoluteTolerance,
vecRelativeTolerance);
TestProblemHandler handler = new TestProblemHandler(pb, integ);
integ.addStepHandler(handler);
integ.integrate(pb,
pb.getInitialTime(), pb.getInitialState(),
pb.getFinalTime(), new double[pb.getDimension()]);
Assert.fail("an exception should have been thrown");
}
@Test
public void testIncreasingTolerance()
{
int previousCalls = Integer.MAX_VALUE;
AdaptiveStepsizeIntegrator integ =
new DormandPrince853Integrator(0, Double.POSITIVE_INFINITY,
Double.NaN, Double.NaN);
for (int i = -12; i < -2; ++i) {
TestProblem1 pb = new TestProblem1();
double minStep = 0;
double maxStep = pb.getFinalTime() - pb.getInitialTime();
double scalAbsoluteTolerance = FastMath.pow(10.0, i);
double scalRelativeTolerance = 0.01 * scalAbsoluteTolerance;
integ.setStepSizeControl(minStep, maxStep, scalAbsoluteTolerance, scalRelativeTolerance);
TestProblemHandler handler = new TestProblemHandler(pb, integ);
integ.addStepHandler(handler);
integ.integrate(pb,
pb.getInitialTime(), pb.getInitialState(),
pb.getFinalTime(), new double[pb.getDimension()]);
// the 1.3 factor is only valid for this test
// and has been obtained from trial and error
// there is no general relation between local and global errors
Assert.assertTrue(handler.getMaximalValueError() < (1.3 * scalAbsoluteTolerance));
Assert.assertEquals(0, handler.getMaximalTimeError(), 1.0e-12);
int calls = pb.getCalls();
Assert.assertEquals(integ.getEvaluations(), calls);
Assert.assertTrue(calls <= previousCalls);
previousCalls = calls;
}
}
@Test
public void testTooLargeFirstStep() {
AdaptiveStepsizeIntegrator integ =
new DormandPrince853Integrator(0, Double.POSITIVE_INFINITY, Double.NaN, Double.NaN);
final double start = 0.0;
final double end = 0.001;
FirstOrderDifferentialEquations equations = new FirstOrderDifferentialEquations() {
public int getDimension() {
return 1;
}
public void computeDerivatives(double t, double[] y, double[] yDot) {
Assert.assertTrue(t >= FastMath.nextAfter(start, Double.NEGATIVE_INFINITY));
Assert.assertTrue(t <= FastMath.nextAfter(end, Double.POSITIVE_INFINITY));
yDot[0] = -100.0 * y[0];
}
};
integ.setStepSizeControl(0, 1.0, 1.0e-6, 1.0e-8);
integ.integrate(equations, start, new double[] { 1.0 }, end, new double[1]);
}
@Test
public void testBackward()
{
TestProblem5 pb = new TestProblem5();
double minStep = 0;
double maxStep = pb.getFinalTime() - pb.getInitialTime();
double scalAbsoluteTolerance = 1.0e-8;
double scalRelativeTolerance = 0.01 * scalAbsoluteTolerance;
FirstOrderIntegrator integ = new DormandPrince853Integrator(minStep, maxStep,
scalAbsoluteTolerance,
scalRelativeTolerance);
TestProblemHandler handler = new TestProblemHandler(pb, integ);
integ.addStepHandler(handler);
integ.integrate(pb, pb.getInitialTime(), pb.getInitialState(),
pb.getFinalTime(), new double[pb.getDimension()]);
Assert.assertTrue(handler.getLastError() < 1.1e-7);
Assert.assertTrue(handler.getMaximalValueError() < 1.1e-7);
Assert.assertEquals(0, handler.getMaximalTimeError(), 1.0e-12);
Assert.assertEquals("Dormand-Prince 8 (5, 3)", integ.getName());
}
@Test
public void testEvents()
{
TestProblem4 pb = new TestProblem4();
double minStep = 0;
double maxStep = pb.getFinalTime() - pb.getInitialTime();
double scalAbsoluteTolerance = 1.0e-9;
double scalRelativeTolerance = 0.01 * scalAbsoluteTolerance;
FirstOrderIntegrator integ = new DormandPrince853Integrator(minStep, maxStep,
scalAbsoluteTolerance,
scalRelativeTolerance);
TestProblemHandler handler = new TestProblemHandler(pb, integ);
integ.addStepHandler(handler);
EventHandler[] functions = pb.getEventsHandlers();
double convergence = 1.0e-8 * maxStep;
for (int l = 0; l < functions.length; ++l) {
integ.addEventHandler(functions[l], Double.POSITIVE_INFINITY, convergence, 1000);
}
Assert.assertEquals(functions.length, integ.getEventHandlers().size());
integ.integrate(pb,
pb.getInitialTime(), pb.getInitialState(),
pb.getFinalTime(), new double[pb.getDimension()]);
Assert.assertEquals(0, handler.getMaximalValueError(), 2.1e-7);
Assert.assertEquals(0, handler.getMaximalTimeError(), convergence);
Assert.assertEquals(12.0, handler.getLastTime(), convergence);
integ.clearEventHandlers();
Assert.assertEquals(0, integ.getEventHandlers().size());
}
@Test
public void testKepler()
{
final TestProblem3 pb = new TestProblem3(0.9);
double minStep = 0;
double maxStep = pb.getFinalTime() - pb.getInitialTime();
double scalAbsoluteTolerance = 1.0e-8;
double scalRelativeTolerance = scalAbsoluteTolerance;
FirstOrderIntegrator integ = new DormandPrince853Integrator(minStep, maxStep,
scalAbsoluteTolerance,
scalRelativeTolerance);
integ.addStepHandler(new KeplerHandler(pb));
integ.integrate(pb,
pb.getInitialTime(), pb.getInitialState(),
pb.getFinalTime(), new double[pb.getDimension()]);
Assert.assertEquals(integ.getEvaluations(), pb.getCalls());
Assert.assertTrue(pb.getCalls() < 3300);
}
@Test
public void testVariableSteps()
{
final TestProblem3 pb = new TestProblem3(0.9);
double minStep = 0;
double maxStep = pb.getFinalTime() - pb.getInitialTime();
double scalAbsoluteTolerance = 1.0e-8;
double scalRelativeTolerance = scalAbsoluteTolerance;
FirstOrderIntegrator integ = new DormandPrince853Integrator(minStep, maxStep,
scalAbsoluteTolerance,
scalRelativeTolerance);
integ.addStepHandler(new VariableHandler());
double stopTime = integ.integrate(pb,
pb.getInitialTime(), pb.getInitialState(),
pb.getFinalTime(), new double[pb.getDimension()]);
Assert.assertEquals(pb.getFinalTime(), stopTime, 1.0e-10);
Assert.assertEquals("Dormand-Prince 8 (5, 3)", integ.getName());
}
@Test
public void testUnstableDerivative()
{
final StepProblem stepProblem = new StepProblem(0.0, 1.0, 2.0);
FirstOrderIntegrator integ =
new DormandPrince853Integrator(0.1, 10, 1.0e-12, 0.0);
integ.addEventHandler(stepProblem, 1.0, 1.0e-12, 1000);
double[] y = { Double.NaN };
integ.integrate(stepProblem, 0.0, new double[] { 0.0 }, 10.0, y);
Assert.assertEquals(8.0, y[0], 1.0e-12);
}
private static class KeplerHandler implements StepHandler {
public KeplerHandler(TestProblem3 pb) {
this.pb = pb;
}
public void init(double t0, double[] y0, double t) {
nbSteps = 0;
maxError = 0;
}
public void handleStep(StepInterpolator interpolator, boolean isLast) {
++nbSteps;
for (int a = 1; a < 10; ++a) {
double prev = interpolator.getPreviousTime();
double curr = interpolator.getCurrentTime();
double interp = ((10 - a) * prev + a * curr) / 10;
interpolator.setInterpolatedTime(interp);
double[] interpolatedY = interpolator.getInterpolatedState ();
double[] theoreticalY = pb.computeTheoreticalState(interpolator.getInterpolatedTime());
double dx = interpolatedY[0] - theoreticalY[0];
double dy = interpolatedY[1] - theoreticalY[1];
double error = dx * dx + dy * dy;
if (error > maxError) {
maxError = error;
}
}
if (isLast) {
Assert.assertTrue(maxError < 2.4e-10);
Assert.assertTrue(nbSteps < 150);
}
}
private int nbSteps;
private double maxError;
private TestProblem3 pb;
}
private static class VariableHandler implements StepHandler {
public VariableHandler() {
firstTime = true;
minStep = 0;
maxStep = 0;
}
public void init(double t0, double[] y0, double t) {
firstTime = true;
minStep = 0;
maxStep = 0;
}
public void handleStep(StepInterpolator interpolator,
boolean isLast) {
double step = FastMath.abs(interpolator.getCurrentTime()
- interpolator.getPreviousTime());
if (firstTime) {
minStep = FastMath.abs(step);
maxStep = minStep;
firstTime = false;
} else {
if (step < minStep) {
minStep = step;
}
if (step > maxStep) {
maxStep = step;
}
}
if (isLast) {
Assert.assertTrue(minStep < (1.0 / 100.0));
Assert.assertTrue(maxStep > (1.0 / 2.0));
}
}
private boolean firstTime = true;
private double minStep = 0;
private double maxStep = 0;
}
} | // You are a professional Java test case writer, please create a test case named `testTooLargeFirstStep` for the issue `Math-MATH-727`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Math-MATH-727
//
// ## Issue-Title:
// too large first step with embedded Runge-Kutta integrators (Dormand-Prince 8(5,3) ...)
//
// ## Issue-Description:
//
// Adaptive step size integrators compute the first step size by themselves if it is not provided.
//
// For embedded Runge-Kutta type, this step size is not checked against the integration range, so if the integration range is extremely short, this step size may evaluate the function out of the range (and in fact it tries afterward to go back, and fails to stop). Gragg-Bulirsch-Stoer integrators do not have this problem, the step size is checked and truncated if needed.
//
//
//
//
//
@Test
public void testTooLargeFirstStep() {
| 202 | 39 | 178 | src/test/java/org/apache/commons/math/ode/nonstiff/DormandPrince853IntegratorTest.java | src/test/java | ```markdown
## Issue-ID: Math-MATH-727
## Issue-Title:
too large first step with embedded Runge-Kutta integrators (Dormand-Prince 8(5,3) ...)
## Issue-Description:
Adaptive step size integrators compute the first step size by themselves if it is not provided.
For embedded Runge-Kutta type, this step size is not checked against the integration range, so if the integration range is extremely short, this step size may evaluate the function out of the range (and in fact it tries afterward to go back, and fails to stop). Gragg-Bulirsch-Stoer integrators do not have this problem, the step size is checked and truncated if needed.
```
You are a professional Java test case writer, please create a test case named `testTooLargeFirstStep` for the issue `Math-MATH-727`, utilizing the provided issue report information and the following function signature.
```java
@Test
public void testTooLargeFirstStep() {
```
| 178 | [
"org.apache.commons.math.ode.nonstiff.EmbeddedRungeKuttaIntegrator"
] | 9187f7a98b6e9614b651ecde27f18c8ad708be8f07484cf81a07c0a723338829 | @Test
public void testTooLargeFirstStep() | // You are a professional Java test case writer, please create a test case named `testTooLargeFirstStep` for the issue `Math-MATH-727`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Math-MATH-727
//
// ## Issue-Title:
// too large first step with embedded Runge-Kutta integrators (Dormand-Prince 8(5,3) ...)
//
// ## Issue-Description:
//
// Adaptive step size integrators compute the first step size by themselves if it is not provided.
//
// For embedded Runge-Kutta type, this step size is not checked against the integration range, so if the integration range is extremely short, this step size may evaluate the function out of the range (and in fact it tries afterward to go back, and fails to stop). Gragg-Bulirsch-Stoer integrators do not have this problem, the step size is checked and truncated if needed.
//
//
//
//
//
| Math | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.math.ode.nonstiff;
import org.apache.commons.math.exception.DimensionMismatchException;
import org.apache.commons.math.exception.NumberIsTooSmallException;
import org.apache.commons.math.ode.FirstOrderDifferentialEquations;
import org.apache.commons.math.ode.FirstOrderIntegrator;
import org.apache.commons.math.ode.TestProblem1;
import org.apache.commons.math.ode.TestProblem3;
import org.apache.commons.math.ode.TestProblem4;
import org.apache.commons.math.ode.TestProblem5;
import org.apache.commons.math.ode.TestProblemHandler;
import org.apache.commons.math.ode.events.EventHandler;
import org.apache.commons.math.ode.sampling.StepHandler;
import org.apache.commons.math.ode.sampling.StepInterpolator;
import org.apache.commons.math.util.FastMath;
import org.junit.Assert;
import org.junit.Test;
public class DormandPrince853IntegratorTest {
@Test
public void testMissedEndEvent() {
final double t0 = 1878250320.0000029;
final double tEvent = 1878250379.9999986;
final double[] k = { 1.0e-4, 1.0e-5, 1.0e-6 };
FirstOrderDifferentialEquations ode = new FirstOrderDifferentialEquations() {
public int getDimension() {
return k.length;
}
public void computeDerivatives(double t, double[] y, double[] yDot) {
for (int i = 0; i < y.length; ++i) {
yDot[i] = k[i] * y[i];
}
}
};
DormandPrince853Integrator integrator = new DormandPrince853Integrator(0.0, 100.0,
1.0e-10, 1.0e-10);
double[] y0 = new double[k.length];
for (int i = 0; i < y0.length; ++i) {
y0[i] = i + 1;
}
double[] y = new double[k.length];
integrator.setInitialStepSize(60.0);
double finalT = integrator.integrate(ode, t0, y0, tEvent, y);
Assert.assertEquals(tEvent, finalT, 5.0e-6);
for (int i = 0; i < y.length; ++i) {
Assert.assertEquals(y0[i] * FastMath.exp(k[i] * (finalT - t0)), y[i], 1.0e-9);
}
integrator.setInitialStepSize(60.0);
integrator.addEventHandler(new EventHandler() {
public void init(double t0, double[] y0, double t) {
}
public void resetState(double t, double[] y) {
}
public double g(double t, double[] y) {
return t - tEvent;
}
public Action eventOccurred(double t, double[] y, boolean increasing) {
Assert.assertEquals(tEvent, t, 5.0e-6);
return Action.CONTINUE;
}
}, Double.POSITIVE_INFINITY, 1.0e-20, 100);
finalT = integrator.integrate(ode, t0, y0, tEvent + 120, y);
Assert.assertEquals(tEvent + 120, finalT, 5.0e-6);
for (int i = 0; i < y.length; ++i) {
Assert.assertEquals(y0[i] * FastMath.exp(k[i] * (finalT - t0)), y[i], 1.0e-9);
}
}
@Test(expected=DimensionMismatchException.class)
public void testDimensionCheck() {
TestProblem1 pb = new TestProblem1();
DormandPrince853Integrator integrator = new DormandPrince853Integrator(0.0, 1.0,
1.0e-10, 1.0e-10);
integrator.integrate(pb,
0.0, new double[pb.getDimension()+10],
1.0, new double[pb.getDimension()+10]);
Assert.fail("an exception should have been thrown");
}
@Test(expected=NumberIsTooSmallException.class)
public void testNullIntervalCheck() {
TestProblem1 pb = new TestProblem1();
DormandPrince853Integrator integrator = new DormandPrince853Integrator(0.0, 1.0,
1.0e-10, 1.0e-10);
integrator.integrate(pb,
0.0, new double[pb.getDimension()],
0.0, new double[pb.getDimension()]);
Assert.fail("an exception should have been thrown");
}
@Test(expected=NumberIsTooSmallException.class)
public void testMinStep() {
TestProblem1 pb = new TestProblem1();
double minStep = 0.1 * (pb.getFinalTime() - pb.getInitialTime());
double maxStep = pb.getFinalTime() - pb.getInitialTime();
double[] vecAbsoluteTolerance = { 1.0e-15, 1.0e-16 };
double[] vecRelativeTolerance = { 1.0e-15, 1.0e-16 };
FirstOrderIntegrator integ = new DormandPrince853Integrator(minStep, maxStep,
vecAbsoluteTolerance,
vecRelativeTolerance);
TestProblemHandler handler = new TestProblemHandler(pb, integ);
integ.addStepHandler(handler);
integ.integrate(pb,
pb.getInitialTime(), pb.getInitialState(),
pb.getFinalTime(), new double[pb.getDimension()]);
Assert.fail("an exception should have been thrown");
}
@Test
public void testIncreasingTolerance()
{
int previousCalls = Integer.MAX_VALUE;
AdaptiveStepsizeIntegrator integ =
new DormandPrince853Integrator(0, Double.POSITIVE_INFINITY,
Double.NaN, Double.NaN);
for (int i = -12; i < -2; ++i) {
TestProblem1 pb = new TestProblem1();
double minStep = 0;
double maxStep = pb.getFinalTime() - pb.getInitialTime();
double scalAbsoluteTolerance = FastMath.pow(10.0, i);
double scalRelativeTolerance = 0.01 * scalAbsoluteTolerance;
integ.setStepSizeControl(minStep, maxStep, scalAbsoluteTolerance, scalRelativeTolerance);
TestProblemHandler handler = new TestProblemHandler(pb, integ);
integ.addStepHandler(handler);
integ.integrate(pb,
pb.getInitialTime(), pb.getInitialState(),
pb.getFinalTime(), new double[pb.getDimension()]);
// the 1.3 factor is only valid for this test
// and has been obtained from trial and error
// there is no general relation between local and global errors
Assert.assertTrue(handler.getMaximalValueError() < (1.3 * scalAbsoluteTolerance));
Assert.assertEquals(0, handler.getMaximalTimeError(), 1.0e-12);
int calls = pb.getCalls();
Assert.assertEquals(integ.getEvaluations(), calls);
Assert.assertTrue(calls <= previousCalls);
previousCalls = calls;
}
}
@Test
public void testTooLargeFirstStep() {
AdaptiveStepsizeIntegrator integ =
new DormandPrince853Integrator(0, Double.POSITIVE_INFINITY, Double.NaN, Double.NaN);
final double start = 0.0;
final double end = 0.001;
FirstOrderDifferentialEquations equations = new FirstOrderDifferentialEquations() {
public int getDimension() {
return 1;
}
public void computeDerivatives(double t, double[] y, double[] yDot) {
Assert.assertTrue(t >= FastMath.nextAfter(start, Double.NEGATIVE_INFINITY));
Assert.assertTrue(t <= FastMath.nextAfter(end, Double.POSITIVE_INFINITY));
yDot[0] = -100.0 * y[0];
}
};
integ.setStepSizeControl(0, 1.0, 1.0e-6, 1.0e-8);
integ.integrate(equations, start, new double[] { 1.0 }, end, new double[1]);
}
@Test
public void testBackward()
{
TestProblem5 pb = new TestProblem5();
double minStep = 0;
double maxStep = pb.getFinalTime() - pb.getInitialTime();
double scalAbsoluteTolerance = 1.0e-8;
double scalRelativeTolerance = 0.01 * scalAbsoluteTolerance;
FirstOrderIntegrator integ = new DormandPrince853Integrator(minStep, maxStep,
scalAbsoluteTolerance,
scalRelativeTolerance);
TestProblemHandler handler = new TestProblemHandler(pb, integ);
integ.addStepHandler(handler);
integ.integrate(pb, pb.getInitialTime(), pb.getInitialState(),
pb.getFinalTime(), new double[pb.getDimension()]);
Assert.assertTrue(handler.getLastError() < 1.1e-7);
Assert.assertTrue(handler.getMaximalValueError() < 1.1e-7);
Assert.assertEquals(0, handler.getMaximalTimeError(), 1.0e-12);
Assert.assertEquals("Dormand-Prince 8 (5, 3)", integ.getName());
}
@Test
public void testEvents()
{
TestProblem4 pb = new TestProblem4();
double minStep = 0;
double maxStep = pb.getFinalTime() - pb.getInitialTime();
double scalAbsoluteTolerance = 1.0e-9;
double scalRelativeTolerance = 0.01 * scalAbsoluteTolerance;
FirstOrderIntegrator integ = new DormandPrince853Integrator(minStep, maxStep,
scalAbsoluteTolerance,
scalRelativeTolerance);
TestProblemHandler handler = new TestProblemHandler(pb, integ);
integ.addStepHandler(handler);
EventHandler[] functions = pb.getEventsHandlers();
double convergence = 1.0e-8 * maxStep;
for (int l = 0; l < functions.length; ++l) {
integ.addEventHandler(functions[l], Double.POSITIVE_INFINITY, convergence, 1000);
}
Assert.assertEquals(functions.length, integ.getEventHandlers().size());
integ.integrate(pb,
pb.getInitialTime(), pb.getInitialState(),
pb.getFinalTime(), new double[pb.getDimension()]);
Assert.assertEquals(0, handler.getMaximalValueError(), 2.1e-7);
Assert.assertEquals(0, handler.getMaximalTimeError(), convergence);
Assert.assertEquals(12.0, handler.getLastTime(), convergence);
integ.clearEventHandlers();
Assert.assertEquals(0, integ.getEventHandlers().size());
}
@Test
public void testKepler()
{
final TestProblem3 pb = new TestProblem3(0.9);
double minStep = 0;
double maxStep = pb.getFinalTime() - pb.getInitialTime();
double scalAbsoluteTolerance = 1.0e-8;
double scalRelativeTolerance = scalAbsoluteTolerance;
FirstOrderIntegrator integ = new DormandPrince853Integrator(minStep, maxStep,
scalAbsoluteTolerance,
scalRelativeTolerance);
integ.addStepHandler(new KeplerHandler(pb));
integ.integrate(pb,
pb.getInitialTime(), pb.getInitialState(),
pb.getFinalTime(), new double[pb.getDimension()]);
Assert.assertEquals(integ.getEvaluations(), pb.getCalls());
Assert.assertTrue(pb.getCalls() < 3300);
}
@Test
public void testVariableSteps()
{
final TestProblem3 pb = new TestProblem3(0.9);
double minStep = 0;
double maxStep = pb.getFinalTime() - pb.getInitialTime();
double scalAbsoluteTolerance = 1.0e-8;
double scalRelativeTolerance = scalAbsoluteTolerance;
FirstOrderIntegrator integ = new DormandPrince853Integrator(minStep, maxStep,
scalAbsoluteTolerance,
scalRelativeTolerance);
integ.addStepHandler(new VariableHandler());
double stopTime = integ.integrate(pb,
pb.getInitialTime(), pb.getInitialState(),
pb.getFinalTime(), new double[pb.getDimension()]);
Assert.assertEquals(pb.getFinalTime(), stopTime, 1.0e-10);
Assert.assertEquals("Dormand-Prince 8 (5, 3)", integ.getName());
}
@Test
public void testUnstableDerivative()
{
final StepProblem stepProblem = new StepProblem(0.0, 1.0, 2.0);
FirstOrderIntegrator integ =
new DormandPrince853Integrator(0.1, 10, 1.0e-12, 0.0);
integ.addEventHandler(stepProblem, 1.0, 1.0e-12, 1000);
double[] y = { Double.NaN };
integ.integrate(stepProblem, 0.0, new double[] { 0.0 }, 10.0, y);
Assert.assertEquals(8.0, y[0], 1.0e-12);
}
private static class KeplerHandler implements StepHandler {
public KeplerHandler(TestProblem3 pb) {
this.pb = pb;
}
public void init(double t0, double[] y0, double t) {
nbSteps = 0;
maxError = 0;
}
public void handleStep(StepInterpolator interpolator, boolean isLast) {
++nbSteps;
for (int a = 1; a < 10; ++a) {
double prev = interpolator.getPreviousTime();
double curr = interpolator.getCurrentTime();
double interp = ((10 - a) * prev + a * curr) / 10;
interpolator.setInterpolatedTime(interp);
double[] interpolatedY = interpolator.getInterpolatedState ();
double[] theoreticalY = pb.computeTheoreticalState(interpolator.getInterpolatedTime());
double dx = interpolatedY[0] - theoreticalY[0];
double dy = interpolatedY[1] - theoreticalY[1];
double error = dx * dx + dy * dy;
if (error > maxError) {
maxError = error;
}
}
if (isLast) {
Assert.assertTrue(maxError < 2.4e-10);
Assert.assertTrue(nbSteps < 150);
}
}
private int nbSteps;
private double maxError;
private TestProblem3 pb;
}
private static class VariableHandler implements StepHandler {
public VariableHandler() {
firstTime = true;
minStep = 0;
maxStep = 0;
}
public void init(double t0, double[] y0, double t) {
firstTime = true;
minStep = 0;
maxStep = 0;
}
public void handleStep(StepInterpolator interpolator,
boolean isLast) {
double step = FastMath.abs(interpolator.getCurrentTime()
- interpolator.getPreviousTime());
if (firstTime) {
minStep = FastMath.abs(step);
maxStep = minStep;
firstTime = false;
} else {
if (step < minStep) {
minStep = step;
}
if (step > maxStep) {
maxStep = step;
}
}
if (isLast) {
Assert.assertTrue(minStep < (1.0 / 100.0));
Assert.assertTrue(maxStep > (1.0 / 2.0));
}
}
private boolean firstTime = true;
private double minStep = 0;
private double maxStep = 0;
}
} |
||
@Test // like using several time the captor in the vararg
public void should_capture_arguments_when_args_count_does_NOT_match() throws Exception {
//given
mock.varargs();
Invocation invocation = getLastInvocation();
//when
InvocationMatcher invocationMatcher = new InvocationMatcher(invocation, (List) asList(new LocalizedMatcher(AnyVararg.ANY_VARARG)));
//then
invocationMatcher.captureArgumentsFrom(invocation);
} | org.mockito.internal.invocation.InvocationMatcherTest::should_capture_arguments_when_args_count_does_NOT_match | test/org/mockito/internal/invocation/InvocationMatcherTest.java | 170 | test/org/mockito/internal/invocation/InvocationMatcherTest.java | should_capture_arguments_when_args_count_does_NOT_match | /*
* Copyright (c) 2007 Mockito contributors
* This program is made available under the terms of the MIT License.
*/
package org.mockito.internal.invocation;
import org.fest.assertions.Assertions;
import org.hamcrest.Matcher;
import org.junit.Before;
import org.junit.Test;
import org.mockito.Mock;
import org.mockito.internal.matchers.*;
import org.mockito.invocation.Invocation;
import org.mockitousage.IMethods;
import org.mockitoutil.TestBase;
import java.lang.reflect.Method;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import static java.util.Arrays.asList;
@SuppressWarnings("unchecked")
public class InvocationMatcherTest extends TestBase {
private InvocationMatcher simpleMethod;
@Mock private IMethods mock;
@Before
public void setup() {
simpleMethod = new InvocationBuilder().mock(mock).simpleMethod().toInvocationMatcher();
}
@Test
public void should_be_a_citizen_of_hashes() throws Exception {
Invocation invocation = new InvocationBuilder().toInvocation();
Invocation invocationTwo = new InvocationBuilder().args("blah").toInvocation();
Map map = new HashMap();
map.put(new InvocationMatcher(invocation), "one");
map.put(new InvocationMatcher(invocationTwo), "two");
assertEquals(2, map.size());
}
@Test
public void should_not_equal_if_number_of_arguments_differ() throws Exception {
InvocationMatcher withOneArg = new InvocationMatcher(new InvocationBuilder().args("test").toInvocation());
InvocationMatcher withTwoArgs = new InvocationMatcher(new InvocationBuilder().args("test", 100).toInvocation());
assertFalse(withOneArg.equals(null));
assertFalse(withOneArg.equals(withTwoArgs));
}
@Test
public void should_to_string_with_matchers() throws Exception {
Matcher m = NotNull.NOT_NULL;
InvocationMatcher notNull = new InvocationMatcher(new InvocationBuilder().toInvocation(), asList(m));
Matcher mTwo = new Equals('x');
InvocationMatcher equals = new InvocationMatcher(new InvocationBuilder().toInvocation(), asList(mTwo));
assertContains("simpleMethod(notNull())", notNull.toString());
assertContains("simpleMethod('x')", equals.toString());
}
@Test
public void should_know_if_is_similar_to() throws Exception {
Invocation same = new InvocationBuilder().mock(mock).simpleMethod().toInvocation();
assertTrue(simpleMethod.hasSimilarMethod(same));
Invocation different = new InvocationBuilder().mock(mock).differentMethod().toInvocation();
assertFalse(simpleMethod.hasSimilarMethod(different));
}
@Test
public void should_not_be_similar_to_verified_invocation() throws Exception {
Invocation verified = new InvocationBuilder().simpleMethod().verified().toInvocation();
assertFalse(simpleMethod.hasSimilarMethod(verified));
}
@Test
public void should_not_be_similar_if_mocks_are_different() throws Exception {
Invocation onDifferentMock = new InvocationBuilder().simpleMethod().mock("different mock").toInvocation();
assertFalse(simpleMethod.hasSimilarMethod(onDifferentMock));
}
@Test
public void should_not_be_similar_if_is_overloaded_but_used_with_the_same_arg() throws Exception {
Method method = IMethods.class.getMethod("simpleMethod", String.class);
Method overloadedMethod = IMethods.class.getMethod("simpleMethod", Object.class);
String sameArg = "test";
InvocationMatcher invocation = new InvocationBuilder().method(method).arg(sameArg).toInvocationMatcher();
Invocation overloadedInvocation = new InvocationBuilder().method(overloadedMethod).arg(sameArg).toInvocation();
assertFalse(invocation.hasSimilarMethod(overloadedInvocation));
}
@Test
public void should_be_similar_if_is_overloaded_but_used_with_different_arg() throws Exception {
Method method = IMethods.class.getMethod("simpleMethod", String.class);
Method overloadedMethod = IMethods.class.getMethod("simpleMethod", Object.class);
InvocationMatcher invocation = new InvocationBuilder().mock(mock).method(method).arg("foo").toInvocationMatcher();
Invocation overloadedInvocation = new InvocationBuilder().mock(mock).method(overloadedMethod).arg("bar").toInvocation();
assertTrue(invocation.hasSimilarMethod(overloadedInvocation));
}
@Test
public void should_capture_arguments_from_invocation() throws Exception {
//given
Invocation invocation = new InvocationBuilder().args("1", 100).toInvocation();
CapturingMatcher capturingMatcher = new CapturingMatcher();
InvocationMatcher invocationMatcher = new InvocationMatcher(invocation, (List) asList(new Equals("1"), capturingMatcher));
//when
invocationMatcher.captureArgumentsFrom(invocation);
//then
assertEquals(1, capturingMatcher.getAllValues().size());
assertEquals(100, capturingMatcher.getLastValue());
}
@Test
public void should_match_varargs_using_any_varargs() throws Exception {
//given
mock.varargs("1", "2");
Invocation invocation = getLastInvocation();
InvocationMatcher invocationMatcher = new InvocationMatcher(invocation, (List) asList(AnyVararg.ANY_VARARG));
//when
boolean match = invocationMatcher.matches(invocation);
//then
assertTrue(match);
}
@Test
public void should_capture_varargs_as_vararg() {}
// Defects4J: flaky method
// @Test
// public void should_capture_varargs_as_vararg() throws Exception {
// //given
// mock.mixedVarargs(1, "a", "b");
// Invocation invocation = getLastInvocation();
// CapturingMatcher m = new CapturingMatcher();
// InvocationMatcher invocationMatcher = new InvocationMatcher(invocation, (List) asList(new Equals(1), new LocalizedMatcher(m)));
//
// //when
// invocationMatcher.captureArgumentsFrom(invocation);
//
// //then
// Assertions.assertThat(m.getAllValues()).containsExactly("a", "b");
// }
@Test // like using several time the captor in the vararg
public void should_capture_arguments_when_args_count_does_NOT_match() throws Exception {
//given
mock.varargs();
Invocation invocation = getLastInvocation();
//when
InvocationMatcher invocationMatcher = new InvocationMatcher(invocation, (List) asList(new LocalizedMatcher(AnyVararg.ANY_VARARG)));
//then
invocationMatcher.captureArgumentsFrom(invocation);
}
@Test
public void should_create_from_invocations() throws Exception {
//given
Invocation i = new InvocationBuilder().toInvocation();
//when
List<InvocationMatcher> out = InvocationMatcher.createFrom(asList(i));
//then
assertEquals(1, out.size());
assertEquals(i, out.get(0).getInvocation());
}
} | // You are a professional Java test case writer, please create a test case named `should_capture_arguments_when_args_count_does_NOT_match` for the issue `Mockito-188`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Mockito-188
//
// ## Issue-Title:
// ArgumentCaptor no longer working for varargs
//
// ## Issue-Description:
// I ran into the issue described here: <http://stackoverflow.com/questions/27303562/why-does-upgrading-mockito-from-1-9-5-to-1-10-8-break-this-captor>
//
//
//
//
@Test // like using several time the captor in the vararg
public void should_capture_arguments_when_args_count_does_NOT_match() throws Exception {
| 170 | // }
// Assertions.assertThat(m.getAllValues()).containsExactly("a", "b");
// //then
//
// invocationMatcher.captureArgumentsFrom(invocation);
// //when
//
// InvocationMatcher invocationMatcher = new InvocationMatcher(invocation, (List) asList(new Equals(1), new LocalizedMatcher(m)));
// CapturingMatcher m = new CapturingMatcher();
// Invocation invocation = getLastInvocation();
// mock.mixedVarargs(1, "a", "b");
// //given
// public void should_capture_varargs_as_vararg() throws Exception {
// @Test
// Defects4J: flaky method | 1 | 159 | test/org/mockito/internal/invocation/InvocationMatcherTest.java | test | ```markdown
## Issue-ID: Mockito-188
## Issue-Title:
ArgumentCaptor no longer working for varargs
## Issue-Description:
I ran into the issue described here: <http://stackoverflow.com/questions/27303562/why-does-upgrading-mockito-from-1-9-5-to-1-10-8-break-this-captor>
```
You are a professional Java test case writer, please create a test case named `should_capture_arguments_when_args_count_does_NOT_match` for the issue `Mockito-188`, utilizing the provided issue report information and the following function signature.
```java
@Test // like using several time the captor in the vararg
public void should_capture_arguments_when_args_count_does_NOT_match() throws Exception {
```
| 159 | [
"org.mockito.internal.invocation.InvocationMatcher"
] | 925630547f73537c27e303a91e766627b827aa0754baf73b406226f25fb9fc8d | @Test // like using several time the captor in the vararg
public void should_capture_arguments_when_args_count_does_NOT_match() throws Exception | // You are a professional Java test case writer, please create a test case named `should_capture_arguments_when_args_count_does_NOT_match` for the issue `Mockito-188`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Mockito-188
//
// ## Issue-Title:
// ArgumentCaptor no longer working for varargs
//
// ## Issue-Description:
// I ran into the issue described here: <http://stackoverflow.com/questions/27303562/why-does-upgrading-mockito-from-1-9-5-to-1-10-8-break-this-captor>
//
//
//
//
| Mockito | /*
* Copyright (c) 2007 Mockito contributors
* This program is made available under the terms of the MIT License.
*/
package org.mockito.internal.invocation;
import org.fest.assertions.Assertions;
import org.hamcrest.Matcher;
import org.junit.Before;
import org.junit.Test;
import org.mockito.Mock;
import org.mockito.internal.matchers.*;
import org.mockito.invocation.Invocation;
import org.mockitousage.IMethods;
import org.mockitoutil.TestBase;
import java.lang.reflect.Method;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import static java.util.Arrays.asList;
@SuppressWarnings("unchecked")
public class InvocationMatcherTest extends TestBase {
private InvocationMatcher simpleMethod;
@Mock private IMethods mock;
@Before
public void setup() {
simpleMethod = new InvocationBuilder().mock(mock).simpleMethod().toInvocationMatcher();
}
@Test
public void should_be_a_citizen_of_hashes() throws Exception {
Invocation invocation = new InvocationBuilder().toInvocation();
Invocation invocationTwo = new InvocationBuilder().args("blah").toInvocation();
Map map = new HashMap();
map.put(new InvocationMatcher(invocation), "one");
map.put(new InvocationMatcher(invocationTwo), "two");
assertEquals(2, map.size());
}
@Test
public void should_not_equal_if_number_of_arguments_differ() throws Exception {
InvocationMatcher withOneArg = new InvocationMatcher(new InvocationBuilder().args("test").toInvocation());
InvocationMatcher withTwoArgs = new InvocationMatcher(new InvocationBuilder().args("test", 100).toInvocation());
assertFalse(withOneArg.equals(null));
assertFalse(withOneArg.equals(withTwoArgs));
}
@Test
public void should_to_string_with_matchers() throws Exception {
Matcher m = NotNull.NOT_NULL;
InvocationMatcher notNull = new InvocationMatcher(new InvocationBuilder().toInvocation(), asList(m));
Matcher mTwo = new Equals('x');
InvocationMatcher equals = new InvocationMatcher(new InvocationBuilder().toInvocation(), asList(mTwo));
assertContains("simpleMethod(notNull())", notNull.toString());
assertContains("simpleMethod('x')", equals.toString());
}
@Test
public void should_know_if_is_similar_to() throws Exception {
Invocation same = new InvocationBuilder().mock(mock).simpleMethod().toInvocation();
assertTrue(simpleMethod.hasSimilarMethod(same));
Invocation different = new InvocationBuilder().mock(mock).differentMethod().toInvocation();
assertFalse(simpleMethod.hasSimilarMethod(different));
}
@Test
public void should_not_be_similar_to_verified_invocation() throws Exception {
Invocation verified = new InvocationBuilder().simpleMethod().verified().toInvocation();
assertFalse(simpleMethod.hasSimilarMethod(verified));
}
@Test
public void should_not_be_similar_if_mocks_are_different() throws Exception {
Invocation onDifferentMock = new InvocationBuilder().simpleMethod().mock("different mock").toInvocation();
assertFalse(simpleMethod.hasSimilarMethod(onDifferentMock));
}
@Test
public void should_not_be_similar_if_is_overloaded_but_used_with_the_same_arg() throws Exception {
Method method = IMethods.class.getMethod("simpleMethod", String.class);
Method overloadedMethod = IMethods.class.getMethod("simpleMethod", Object.class);
String sameArg = "test";
InvocationMatcher invocation = new InvocationBuilder().method(method).arg(sameArg).toInvocationMatcher();
Invocation overloadedInvocation = new InvocationBuilder().method(overloadedMethod).arg(sameArg).toInvocation();
assertFalse(invocation.hasSimilarMethod(overloadedInvocation));
}
@Test
public void should_be_similar_if_is_overloaded_but_used_with_different_arg() throws Exception {
Method method = IMethods.class.getMethod("simpleMethod", String.class);
Method overloadedMethod = IMethods.class.getMethod("simpleMethod", Object.class);
InvocationMatcher invocation = new InvocationBuilder().mock(mock).method(method).arg("foo").toInvocationMatcher();
Invocation overloadedInvocation = new InvocationBuilder().mock(mock).method(overloadedMethod).arg("bar").toInvocation();
assertTrue(invocation.hasSimilarMethod(overloadedInvocation));
}
@Test
public void should_capture_arguments_from_invocation() throws Exception {
//given
Invocation invocation = new InvocationBuilder().args("1", 100).toInvocation();
CapturingMatcher capturingMatcher = new CapturingMatcher();
InvocationMatcher invocationMatcher = new InvocationMatcher(invocation, (List) asList(new Equals("1"), capturingMatcher));
//when
invocationMatcher.captureArgumentsFrom(invocation);
//then
assertEquals(1, capturingMatcher.getAllValues().size());
assertEquals(100, capturingMatcher.getLastValue());
}
@Test
public void should_match_varargs_using_any_varargs() throws Exception {
//given
mock.varargs("1", "2");
Invocation invocation = getLastInvocation();
InvocationMatcher invocationMatcher = new InvocationMatcher(invocation, (List) asList(AnyVararg.ANY_VARARG));
//when
boolean match = invocationMatcher.matches(invocation);
//then
assertTrue(match);
}
@Test
public void should_capture_varargs_as_vararg() {}
// Defects4J: flaky method
// @Test
// public void should_capture_varargs_as_vararg() throws Exception {
// //given
// mock.mixedVarargs(1, "a", "b");
// Invocation invocation = getLastInvocation();
// CapturingMatcher m = new CapturingMatcher();
// InvocationMatcher invocationMatcher = new InvocationMatcher(invocation, (List) asList(new Equals(1), new LocalizedMatcher(m)));
//
// //when
// invocationMatcher.captureArgumentsFrom(invocation);
//
// //then
// Assertions.assertThat(m.getAllValues()).containsExactly("a", "b");
// }
@Test // like using several time the captor in the vararg
public void should_capture_arguments_when_args_count_does_NOT_match() throws Exception {
//given
mock.varargs();
Invocation invocation = getLastInvocation();
//when
InvocationMatcher invocationMatcher = new InvocationMatcher(invocation, (List) asList(new LocalizedMatcher(AnyVararg.ANY_VARARG)));
//then
invocationMatcher.captureArgumentsFrom(invocation);
}
@Test
public void should_create_from_invocations() throws Exception {
//given
Invocation i = new InvocationBuilder().toInvocation();
//when
List<InvocationMatcher> out = InvocationMatcher.createFrom(asList(i));
//then
assertEquals(1, out.size());
assertEquals(i, out.get(0).getInvocation());
}
} |
|
public void testBackwardsInferenceNew() {
inFunction(
"/**\n" +
" * @constructor\n" +
" * @param {{foo: (number|undefined)}} x\n" +
" */" +
"function F(x) {}" +
"var y = {};" +
"new F(y);");
assertEquals("{foo: (number|undefined)}", getType("y").toString());
} | com.google.javascript.jscomp.TypeInferenceTest::testBackwardsInferenceNew | test/com/google/javascript/jscomp/TypeInferenceTest.java | 890 | test/com/google/javascript/jscomp/TypeInferenceTest.java | testBackwardsInferenceNew | /*
* Copyright 2008 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import static com.google.javascript.rhino.jstype.JSTypeNative.ALL_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.ARRAY_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.BOOLEAN_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.FUNCTION_INSTANCE_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.NULL_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.NUMBER_OBJECT_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.NUMBER_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.OBJECT_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.STRING_OBJECT_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.STRING_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.UNKNOWN_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.VOID_TYPE;
import com.google.common.base.Joiner;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.Maps;
import com.google.javascript.jscomp.CodingConvention.AssertionFunctionSpec;
import com.google.javascript.jscomp.CompilerOptions.LanguageMode;
import com.google.javascript.jscomp.DataFlowAnalysis.BranchedFlowState;
import com.google.javascript.jscomp.type.FlowScope;
import com.google.javascript.jscomp.type.ReverseAbstractInterpreter;
import com.google.javascript.jscomp.type.SemanticReverseAbstractInterpreter;
import com.google.javascript.rhino.Node;
import com.google.javascript.rhino.jstype.EnumType;
import com.google.javascript.rhino.jstype.JSType;
import com.google.javascript.rhino.jstype.JSTypeNative;
import com.google.javascript.rhino.jstype.JSTypeRegistry;
import com.google.javascript.rhino.jstype.StaticSlot;
import com.google.javascript.rhino.testing.Asserts;
import junit.framework.TestCase;
import java.util.Map;
/**
* Tests {@link TypeInference}.
*
*/
public class TypeInferenceTest extends TestCase {
private Compiler compiler;
private JSTypeRegistry registry;
private Map<String,JSType> assumptions;
private FlowScope returnScope;
private static final Map<String, AssertionFunctionSpec>
ASSERTION_FUNCTION_MAP = Maps.newHashMap();
static {
for (AssertionFunctionSpec func :
new ClosureCodingConvention().getAssertionFunctions()) {
ASSERTION_FUNCTION_MAP.put(func.getFunctionName(), func);
}
}
@Override
public void setUp() {
compiler = new Compiler();
CompilerOptions options = new CompilerOptions();
options.setLanguageIn(LanguageMode.ECMASCRIPT5);
compiler.initOptions(options);
registry = compiler.getTypeRegistry();
assumptions = Maps.newHashMap();
returnScope = null;
}
private void assuming(String name, JSType type) {
assumptions.put(name, type);
}
private void assuming(String name, JSTypeNative type) {
assuming(name, registry.getNativeType(type));
}
private void inFunction(String js) {
// Parse the body of the function.
Node root = compiler.parseTestCode("(function() {" + js + "});");
assertEquals("parsing error: " +
Joiner.on(", ").join(compiler.getErrors()),
0, compiler.getErrorCount());
Node n = root.getFirstChild().getFirstChild();
// Create the scope with the assumptions.
TypedScopeCreator scopeCreator = new TypedScopeCreator(compiler);
Scope assumedScope = scopeCreator.createScope(
n, scopeCreator.createScope(root, null));
for (Map.Entry<String,JSType> entry : assumptions.entrySet()) {
assumedScope.declare(entry.getKey(), null, entry.getValue(), null);
}
// Create the control graph.
ControlFlowAnalysis cfa = new ControlFlowAnalysis(compiler, false, false);
cfa.process(null, n);
ControlFlowGraph<Node> cfg = cfa.getCfg();
// Create a simple reverse abstract interpreter.
ReverseAbstractInterpreter rai = new SemanticReverseAbstractInterpreter(
compiler.getCodingConvention(), registry);
// Do the type inference by data-flow analysis.
TypeInference dfa = new TypeInference(compiler, cfg, rai, assumedScope,
ASSERTION_FUNCTION_MAP);
dfa.analyze();
// Get the scope of the implicit return.
BranchedFlowState<FlowScope> rtnState =
cfg.getImplicitReturn().getAnnotation();
returnScope = rtnState.getIn();
}
private JSType getType(String name) {
assertTrue("The return scope should not be null.", returnScope != null);
StaticSlot<JSType> var = returnScope.getSlot(name);
assertTrue("The variable " + name + " is missing from the scope.",
var != null);
return var.getType();
}
private void verify(String name, JSType type) {
Asserts.assertTypeEquals(type, getType(name));
}
private void verify(String name, JSTypeNative type) {
verify(name, registry.getNativeType(type));
}
private void verifySubtypeOf(String name, JSType type) {
JSType varType = getType(name);
assertTrue("The variable " + name + " is missing a type.", varType != null);
assertTrue("The type " + varType + " of variable " + name +
" is not a subtype of " + type +".", varType.isSubtype(type));
}
private void verifySubtypeOf(String name, JSTypeNative type) {
verifySubtypeOf(name, registry.getNativeType(type));
}
private EnumType createEnumType(String name, JSTypeNative elemType) {
return createEnumType(name, registry.getNativeType(elemType));
}
private EnumType createEnumType(String name, JSType elemType) {
return registry.createEnumType(name, null, elemType);
}
private JSType createUndefinableType(JSTypeNative type) {
return registry.createUnionType(
registry.getNativeType(type), registry.getNativeType(VOID_TYPE));
}
private JSType createNullableType(JSTypeNative type) {
return createNullableType(registry.getNativeType(type));
}
private JSType createNullableType(JSType type) {
return registry.createNullableType(type);
}
private JSType createUnionType(JSTypeNative type1, JSTypeNative type2) {
return registry.createUnionType(
registry.getNativeType(type1), registry.getNativeType(type2));
}
public void testAssumption() {
assuming("x", NUMBER_TYPE);
inFunction("");
verify("x", NUMBER_TYPE);
}
public void testVar() {
inFunction("var x = 1;");
verify("x", NUMBER_TYPE);
}
public void testEmptyVar() {
inFunction("var x;");
verify("x", VOID_TYPE);
}
public void testAssignment() {
assuming("x", OBJECT_TYPE);
inFunction("x = 1;");
verify("x", NUMBER_TYPE);
}
public void testGetProp() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("x.y();");
verify("x", OBJECT_TYPE);
}
public void testGetElemDereference() {
assuming("x", createUndefinableType(OBJECT_TYPE));
inFunction("x['z'] = 3;");
verify("x", OBJECT_TYPE);
}
public void testIf1() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = {}; if (x) { y = x; }");
verifySubtypeOf("y", OBJECT_TYPE);
}
public void testIf1a() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = {}; if (x != null) { y = x; }");
verifySubtypeOf("y", OBJECT_TYPE);
}
public void testIf2() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = x; if (x) { y = x; } else { y = {}; }");
verifySubtypeOf("y", OBJECT_TYPE);
}
public void testIf3() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = 1; if (x) { y = x; }");
verify("y", createUnionType(OBJECT_TYPE, NUMBER_TYPE));
}
public void testAssert1() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assert(x); out2 = x;");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
}
public void testAssert1a() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assert(x !== null); out2 = x;");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
}
public void testAssert2() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
inFunction("goog.asserts.assert(1, x); out1 = x;");
verify("out1", startType);
}
public void testAssert3() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
assuming("y", startType);
inFunction("out1 = x; goog.asserts.assert(x && y); out2 = x; out3 = y;");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
verify("out3", OBJECT_TYPE);
}
public void testAssert4() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
assuming("y", startType);
inFunction("out1 = x; goog.asserts.assert(x && !y); out2 = x; out3 = y;");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
verify("out3", NULL_TYPE);
}
public void testAssert5() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
assuming("y", startType);
inFunction("goog.asserts.assert(x || y); out1 = x; out2 = y;");
verify("out1", startType);
verify("out2", startType);
}
public void testAssert6() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x.y", startType);
inFunction("out1 = x.y; goog.asserts.assert(x.y); out2 = x.y;");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
}
public void testAssert7() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
inFunction("out1 = x; out2 = goog.asserts.assert(x);");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
}
public void testAssert8() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
inFunction("out1 = x; out2 = goog.asserts.assert(x != null);");
verify("out1", startType);
verify("out2", BOOLEAN_TYPE);
}
public void testAssert9() {
JSType startType = createNullableType(NUMBER_TYPE);
assuming("x", startType);
inFunction("out1 = x; out2 = goog.asserts.assert(y = x);");
verify("out1", startType);
verify("out2", NUMBER_TYPE);
}
public void testAssert10() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
assuming("y", startType);
inFunction("out1 = x; out2 = goog.asserts.assert(x && y); out3 = x;");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
verify("out3", OBJECT_TYPE);
}
public void testAssertNumber() {
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertNumber(x); out2 = x;");
verify("out1", startType);
verify("out2", NUMBER_TYPE);
}
public void testAssertNumber2() {
// Make sure it ignores expressions.
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("goog.asserts.assertNumber(x + x); out1 = x;");
verify("out1", startType);
}
public void testAssertNumber3() {
// Make sure it ignores expressions.
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("out1 = x; out2 = goog.asserts.assertNumber(x + x);");
verify("out1", startType);
verify("out2", NUMBER_TYPE);
}
public void testAssertString() {
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertString(x); out2 = x;");
verify("out1", startType);
verify("out2", STRING_TYPE);
}
public void testAssertFunction() {
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertFunction(x); out2 = x;");
verify("out1", startType);
verifySubtypeOf("out2", FUNCTION_INSTANCE_TYPE);
}
public void testAssertObject() {
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertObject(x); out2 = x;");
verify("out1", startType);
verifySubtypeOf("out2", OBJECT_TYPE);
}
public void testAssertObject2() {
JSType startType = createNullableType(ARRAY_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertObject(x); out2 = x;");
verify("out1", startType);
verify("out2", ARRAY_TYPE);
}
public void testAssertObject3() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x.y", startType);
inFunction("out1 = x.y; goog.asserts.assertObject(x.y); out2 = x.y;");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
}
public void testAssertObject4() {
JSType startType = createNullableType(ARRAY_TYPE);
assuming("x", startType);
inFunction("out1 = x; out2 = goog.asserts.assertObject(x);");
verify("out1", startType);
verify("out2", ARRAY_TYPE);
}
public void testAssertObject5() {
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction(
"out1 = x;" +
"out2 = /** @type {!Array} */ (goog.asserts.assertObject(x));");
verify("out1", startType);
verify("out2", ARRAY_TYPE);
}
public void testAssertArray() {
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertArray(x); out2 = x;");
verify("out1", startType);
verifySubtypeOf("out2", ARRAY_TYPE);
}
public void testAssertInstanceof() {
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertInstanceof(x); out2 = x;");
verify("out1", startType);
verifySubtypeOf("out2", OBJECT_TYPE);
}
public void testReturn1() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("if (x) { return x; }\nx = {};\nreturn x;");
verify("x", OBJECT_TYPE);
}
public void testReturn2() {
assuming("x", createNullableType(NUMBER_TYPE));
inFunction("if (!x) { x = 0; }\nreturn x;");
verify("x", NUMBER_TYPE);
}
public void testWhile1() {
assuming("x", createNullableType(NUMBER_TYPE));
inFunction("while (!x) { if (x == null) { x = 0; } else { x = 1; } }");
verify("x", NUMBER_TYPE);
}
public void testWhile2() {
assuming("x", createNullableType(NUMBER_TYPE));
inFunction("while (!x) { x = {}; }");
verifySubtypeOf("x", createUnionType(OBJECT_TYPE, NUMBER_TYPE));
}
public void testDo() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("do { x = 1; } while (!x);");
verify("x", NUMBER_TYPE);
}
public void testFor1() {
assuming("y", NUMBER_TYPE);
inFunction("var x = null; var i = null; for (i=y; !i; i=1) { x = 1; }");
verify("x", createNullableType(NUMBER_TYPE));
verify("i", NUMBER_TYPE);
}
public void testFor2() {
assuming("y", OBJECT_TYPE);
inFunction("var x = null; var i = null; for (i in y) { x = 1; }");
verify("x", createNullableType(NUMBER_TYPE));
verify("i", createNullableType(STRING_TYPE));
}
public void testFor3() {
assuming("y", OBJECT_TYPE);
inFunction("var x = null; var i = null; for (var i in y) { x = 1; }");
verify("x", createNullableType(NUMBER_TYPE));
verify("i", createNullableType(STRING_TYPE));
}
public void testFor4() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = {};\n" +
"if (x) { for (var i = 0; i < 10; i++) { break; } y = x; }");
verifySubtypeOf("y", OBJECT_TYPE);
}
public void testSwitch1() {
assuming("x", NUMBER_TYPE);
inFunction("var y = null; switch(x) {\n" +
"case 1: y = 1; break;\n" +
"case 2: y = {};\n" +
"case 3: y = {};\n" +
"default: y = 0;}");
verify("y", NUMBER_TYPE);
}
public void testSwitch2() {
assuming("x", ALL_TYPE);
inFunction("var y = null; switch (typeof x) {\n" +
"case 'string':\n" +
" y = x;\n" +
" return;" +
"default:\n" +
" y = 'a';\n" +
"}");
verify("y", STRING_TYPE);
}
public void testSwitch3() {
assuming("x",
createNullableType(createUnionType(NUMBER_TYPE, STRING_TYPE)));
inFunction("var y; var z; switch (typeof x) {\n" +
"case 'string':\n" +
" y = 1; z = null;\n" +
" return;\n" +
"case 'number':\n" +
" y = x; z = null;\n" +
" return;" +
"default:\n" +
" y = 1; z = x;\n" +
"}");
verify("y", NUMBER_TYPE);
verify("z", NULL_TYPE);
}
public void testSwitch4() {
assuming("x", ALL_TYPE);
inFunction("var y = null; switch (typeof x) {\n" +
"case 'string':\n" +
"case 'number':\n" +
" y = x;\n" +
" return;\n" +
"default:\n" +
" y = 1;\n" +
"}\n");
verify("y", createUnionType(NUMBER_TYPE, STRING_TYPE));
}
public void testCall1() {
assuming("x",
createNullableType(
registry.createFunctionType(registry.getNativeType(NUMBER_TYPE))));
inFunction("var y = x();");
verify("y", NUMBER_TYPE);
}
public void testNew1() {
assuming("x",
createNullableType(
registry.getNativeType(JSTypeNative.U2U_CONSTRUCTOR_TYPE)));
inFunction("var y = new x();");
verify("y", JSTypeNative.NO_OBJECT_TYPE);
}
public void testInnerFunction1() {
inFunction("var x = 1; function f() { x = null; };");
verify("x", NUMBER_TYPE);
}
public void testInnerFunction2() {
inFunction("var x = 1; var f = function() { x = null; };");
verify("x", NUMBER_TYPE);
}
public void testHook() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = x ? x : {};");
verifySubtypeOf("y", OBJECT_TYPE);
}
public void testThrow() {
assuming("x", createNullableType(NUMBER_TYPE));
inFunction("var y = 1;\n" +
"if (x == null) { throw new Error('x is null') }\n" +
"y = x;");
verify("y", NUMBER_TYPE);
}
public void testTry1() {
assuming("x", NUMBER_TYPE);
inFunction("var y = null; try { y = null; } finally { y = x; }");
verify("y", NUMBER_TYPE);
}
public void testTry2() {
assuming("x", NUMBER_TYPE);
inFunction("var y = null;\n" +
"try { } catch (e) { y = null; } finally { y = x; }");
verify("y", NUMBER_TYPE);
}
public void testTry3() {
assuming("x", NUMBER_TYPE);
inFunction("var y = null; try { y = x; } catch (e) { }");
verify("y", NUMBER_TYPE);
}
public void testCatch1() {
inFunction("var y = null; try { foo(); } catch (e) { y = e; }");
verify("y", UNKNOWN_TYPE);
}
public void testCatch2() {
inFunction("var y = null; var e = 3; try { foo(); } catch (e) { y = e; }");
verify("y", UNKNOWN_TYPE);
}
public void testUnknownType1() {
inFunction("var y = 3; y = x;");
verify("y", UNKNOWN_TYPE);
}
public void testUnknownType2() {
assuming("x", ARRAY_TYPE);
inFunction("var y = 5; y = x[0];");
verify("y", UNKNOWN_TYPE);
}
public void testInfiniteLoop1() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("x = {}; while(x != null) { x = {}; }");
}
public void testInfiniteLoop2() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("x = {}; do { x = null; } while (x == null);");
}
public void testJoin1() {
JSType unknownOrNull = createUnionType(NULL_TYPE, UNKNOWN_TYPE);
assuming("x", BOOLEAN_TYPE);
assuming("unknownOrNull", unknownOrNull);
inFunction("var y; if (x) y = unknownOrNull; else y = null;");
verify("y", unknownOrNull);
}
public void testJoin2() {
JSType unknownOrNull = createUnionType(NULL_TYPE, UNKNOWN_TYPE);
assuming("x", BOOLEAN_TYPE);
assuming("unknownOrNull", unknownOrNull);
inFunction("var y; if (x) y = null; else y = unknownOrNull;");
verify("y", unknownOrNull);
}
public void testArrayLit() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = 3; if (x) { x = [y = x]; }");
verify("x", createUnionType(NULL_TYPE, ARRAY_TYPE));
verify("y", createUnionType(NUMBER_TYPE, OBJECT_TYPE));
}
public void testGetElem() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = 3; if (x) { x = x[y = x]; }");
verify("x", UNKNOWN_TYPE);
verify("y", createUnionType(NUMBER_TYPE, OBJECT_TYPE));
}
public void testEnumRAI1() {
JSType enumType = createEnumType("MyEnum", ARRAY_TYPE).getElementsType();
assuming("x", enumType);
inFunction("var y = null; if (x) y = x;");
verify("y", createNullableType(enumType));
}
public void testEnumRAI2() {
JSType enumType = createEnumType("MyEnum", NUMBER_TYPE).getElementsType();
assuming("x", enumType);
inFunction("var y = null; if (typeof x == 'number') y = x;");
verify("y", createNullableType(enumType));
}
public void testEnumRAI3() {
JSType enumType = createEnumType("MyEnum", NUMBER_TYPE).getElementsType();
assuming("x", enumType);
inFunction("var y = null; if (x && typeof x == 'number') y = x;");
verify("y", createNullableType(enumType));
}
public void testEnumRAI4() {
JSType enumType = createEnumType("MyEnum",
createUnionType(STRING_TYPE, NUMBER_TYPE)).getElementsType();
assuming("x", enumType);
inFunction("var y = null; if (typeof x == 'number') y = x;");
verify("y", createNullableType(NUMBER_TYPE));
}
public void testShortCircuitingAnd() {
assuming("x", NUMBER_TYPE);
inFunction("var y = null; if (x && (y = 3)) { }");
verify("y", createNullableType(NUMBER_TYPE));
}
public void testShortCircuitingAnd2() {
assuming("x", NUMBER_TYPE);
inFunction("var y = null; var z = 4; if (x && (y = 3)) { z = y; }");
verify("z", NUMBER_TYPE);
}
public void testShortCircuitingOr() {
assuming("x", NUMBER_TYPE);
inFunction("var y = null; if (x || (y = 3)) { }");
verify("y", createNullableType(NUMBER_TYPE));
}
public void testShortCircuitingOr2() {
assuming("x", NUMBER_TYPE);
inFunction("var y = null; var z = 4; if (x || (y = 3)) { z = y; }");
verify("z", createNullableType(NUMBER_TYPE));
}
public void testAssignInCondition() {
assuming("x", createNullableType(NUMBER_TYPE));
inFunction("var y; if (!(y = x)) { y = 3; }");
verify("y", NUMBER_TYPE);
}
public void testInstanceOf1() {
assuming("x", OBJECT_TYPE);
inFunction("var y = null; if (x instanceof String) y = x;");
verify("y", createNullableType(STRING_OBJECT_TYPE));
}
public void testInstanceOf2() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = 1; if (x instanceof String) y = x;");
verify("y", createUnionType(STRING_OBJECT_TYPE, NUMBER_TYPE));
}
public void testInstanceOf3() {
assuming("x", createUnionType(STRING_OBJECT_TYPE, NUMBER_OBJECT_TYPE));
inFunction("var y = null; if (x instanceof String) y = x;");
verify("y", createNullableType(STRING_OBJECT_TYPE));
}
public void testInstanceOf4() {
assuming("x", createUnionType(STRING_OBJECT_TYPE, NUMBER_OBJECT_TYPE));
inFunction("var y = null; if (x instanceof String); else y = x;");
verify("y", createNullableType(NUMBER_OBJECT_TYPE));
}
public void testInstanceOf5() {
assuming("x", OBJECT_TYPE);
inFunction("var y = null; if (x instanceof String); else y = x;");
verify("y", createNullableType(OBJECT_TYPE));
}
public void testFlattening() {
for (int i = 0; i < LinkedFlowScope.MAX_DEPTH + 1; i++) {
assuming("s" + i, ALL_TYPE);
}
assuming("b", JSTypeNative.BOOLEAN_TYPE);
StringBuilder body = new StringBuilder();
body.append("if (b) {");
for (int i = 0; i < LinkedFlowScope.MAX_DEPTH + 1; i++) {
body.append("s");
body.append(i);
body.append(" = 1;\n");
}
body.append(" } else { ");
for (int i = 0; i < LinkedFlowScope.MAX_DEPTH + 1; i++) {
body.append("s");
body.append(i);
body.append(" = 'ONE';\n");
}
body.append("}");
JSType numberORString = createUnionType(NUMBER_TYPE, STRING_TYPE);
inFunction(body.toString());
for (int i = 0; i < LinkedFlowScope.MAX_DEPTH + 1; i++) {
verify("s" + i, numberORString);
}
}
public void testUnary() {
assuming("x", NUMBER_TYPE);
inFunction("var y = +x;");
verify("y", NUMBER_TYPE);
inFunction("var z = -x;");
verify("z", NUMBER_TYPE);
}
public void testAdd1() {
assuming("x", NUMBER_TYPE);
inFunction("var y = x + 5;");
verify("y", NUMBER_TYPE);
}
public void testAdd2() {
assuming("x", NUMBER_TYPE);
inFunction("var y = x + '5';");
verify("y", STRING_TYPE);
}
public void testAdd3() {
assuming("x", NUMBER_TYPE);
inFunction("var y = '5' + x;");
verify("y", STRING_TYPE);
}
public void testAssignAdd() {
assuming("x", NUMBER_TYPE);
inFunction("x += '5';");
verify("x", STRING_TYPE);
}
public void testComparison() {
inFunction("var x = 'foo'; var y = (x = 3) < 4;");
verify("x", NUMBER_TYPE);
inFunction("var x = 'foo'; var y = (x = 3) > 4;");
verify("x", NUMBER_TYPE);
inFunction("var x = 'foo'; var y = (x = 3) <= 4;");
verify("x", NUMBER_TYPE);
inFunction("var x = 'foo'; var y = (x = 3) >= 4;");
verify("x", NUMBER_TYPE);
}
public void testThrownExpression() {
inFunction("var x = 'foo'; "
+ "try { throw new Error(x = 3); } catch (ex) {}");
verify("x", NUMBER_TYPE);
}
public void testObjectLit() {
inFunction("var x = {}; var out = x.a;");
verify("out", UNKNOWN_TYPE); // Shouldn't this be 'undefined'?
inFunction("var x = {a:1}; var out = x.a;");
verify("out", NUMBER_TYPE);
inFunction("var x = {a:1}; var out = x.a; x.a = 'string'; var out2 = x.a;");
verify("out", NUMBER_TYPE);
verify("out2", STRING_TYPE);
inFunction("var x = { get a() {return 1} }; var out = x.a;");
verify("out", UNKNOWN_TYPE);
inFunction(
"var x = {" +
" /** @return {number} */ get a() {return 1}" +
"};" +
"var out = x.a;");
verify("out", NUMBER_TYPE);
inFunction("var x = { set a(b) {} }; var out = x.a;");
verify("out", UNKNOWN_TYPE);
inFunction("var x = { " +
"/** @param {number} b */ set a(b) {} };" +
"var out = x.a;");
verify("out", NUMBER_TYPE);
}
public void testCast1() {
inFunction("var x = /** @type {Object} */ (this);");
verify("x", createNullableType(OBJECT_TYPE));
}
public void testCast2() {
inFunction(
"/** @return {boolean} */" +
"Object.prototype.method = function() { return true; };" +
"var x = /** @type {Object} */ (this).method;");
verify(
"x",
registry.createFunctionType(
registry.getNativeObjectType(OBJECT_TYPE),
registry.getNativeType(BOOLEAN_TYPE),
ImmutableList.<JSType>of() /* params */));
}
public void testBackwardsInferenceCall() {
inFunction(
"/** @param {{foo: (number|undefined)}} x */" +
"function f(x) {}" +
"var y = {};" +
"f(y);");
assertEquals("{foo: (number|undefined)}", getType("y").toString());
}
public void testBackwardsInferenceNew() {
inFunction(
"/**\n" +
" * @constructor\n" +
" * @param {{foo: (number|undefined)}} x\n" +
" */" +
"function F(x) {}" +
"var y = {};" +
"new F(y);");
assertEquals("{foo: (number|undefined)}", getType("y").toString());
}
} | // You are a professional Java test case writer, please create a test case named `testBackwardsInferenceNew` for the issue `Closure-729`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-729
//
// ## Issue-Title:
// anonymous object type inference behavior is different when calling constructors
//
// ## Issue-Description:
// The following compiles fine with:
// java -jar build/compiler.jar --compilation\_level=ADVANCED\_OPTIMIZATIONS --jscomp\_error=accessControls --jscomp\_error=checkTypes --jscomp\_error=checkVars --js ~/Desktop/reverse.js
//
// reverse.js:
// /\*\*
// \* @param {{prop1: string, prop2: (number|undefined)}} parry
// \*/
// function callz(parry) {
// if (parry.prop2 && parry.prop2 < 5) alert('alright!');
// alert(parry.prop1);
// }
//
// callz({prop1: 'hi'});
//
//
//
// However, the following does not:
// /\*\*
// \* @param {{prop1: string, prop2: (number|undefined)}} parry
// \* @constructor
// \*/
// function callz(parry) {
// if (parry.prop2 && parry.prop2 < 5) alert('alright!');
// alert(parry.prop1);
// }
//
// new callz({prop1: 'hi'});
//
//
// /Users/dolapo/Desktop/reverse.js:10: ERROR - actual parameter 1 of callz does not match formal parameter
// found : {prop1: string}
// required: {prop1: string, prop2: (number|undefined)}
// new callz({prop1: 'hi'});
//
//
//
// Thanks!
//
//
public void testBackwardsInferenceNew() {
| 890 | 25 | 879 | test/com/google/javascript/jscomp/TypeInferenceTest.java | test | ```markdown
## Issue-ID: Closure-729
## Issue-Title:
anonymous object type inference behavior is different when calling constructors
## Issue-Description:
The following compiles fine with:
java -jar build/compiler.jar --compilation\_level=ADVANCED\_OPTIMIZATIONS --jscomp\_error=accessControls --jscomp\_error=checkTypes --jscomp\_error=checkVars --js ~/Desktop/reverse.js
reverse.js:
/\*\*
\* @param {{prop1: string, prop2: (number|undefined)}} parry
\*/
function callz(parry) {
if (parry.prop2 && parry.prop2 < 5) alert('alright!');
alert(parry.prop1);
}
callz({prop1: 'hi'});
However, the following does not:
/\*\*
\* @param {{prop1: string, prop2: (number|undefined)}} parry
\* @constructor
\*/
function callz(parry) {
if (parry.prop2 && parry.prop2 < 5) alert('alright!');
alert(parry.prop1);
}
new callz({prop1: 'hi'});
/Users/dolapo/Desktop/reverse.js:10: ERROR - actual parameter 1 of callz does not match formal parameter
found : {prop1: string}
required: {prop1: string, prop2: (number|undefined)}
new callz({prop1: 'hi'});
Thanks!
```
You are a professional Java test case writer, please create a test case named `testBackwardsInferenceNew` for the issue `Closure-729`, utilizing the provided issue report information and the following function signature.
```java
public void testBackwardsInferenceNew() {
```
| 879 | [
"com.google.javascript.jscomp.TypeInference"
] | 9256fab7e6a6f61e23d98b65f0f3520a5bff477a00e71f94ccc1310167761262 | public void testBackwardsInferenceNew() | // You are a professional Java test case writer, please create a test case named `testBackwardsInferenceNew` for the issue `Closure-729`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-729
//
// ## Issue-Title:
// anonymous object type inference behavior is different when calling constructors
//
// ## Issue-Description:
// The following compiles fine with:
// java -jar build/compiler.jar --compilation\_level=ADVANCED\_OPTIMIZATIONS --jscomp\_error=accessControls --jscomp\_error=checkTypes --jscomp\_error=checkVars --js ~/Desktop/reverse.js
//
// reverse.js:
// /\*\*
// \* @param {{prop1: string, prop2: (number|undefined)}} parry
// \*/
// function callz(parry) {
// if (parry.prop2 && parry.prop2 < 5) alert('alright!');
// alert(parry.prop1);
// }
//
// callz({prop1: 'hi'});
//
//
//
// However, the following does not:
// /\*\*
// \* @param {{prop1: string, prop2: (number|undefined)}} parry
// \* @constructor
// \*/
// function callz(parry) {
// if (parry.prop2 && parry.prop2 < 5) alert('alright!');
// alert(parry.prop1);
// }
//
// new callz({prop1: 'hi'});
//
//
// /Users/dolapo/Desktop/reverse.js:10: ERROR - actual parameter 1 of callz does not match formal parameter
// found : {prop1: string}
// required: {prop1: string, prop2: (number|undefined)}
// new callz({prop1: 'hi'});
//
//
//
// Thanks!
//
//
| Closure | /*
* Copyright 2008 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import static com.google.javascript.rhino.jstype.JSTypeNative.ALL_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.ARRAY_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.BOOLEAN_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.FUNCTION_INSTANCE_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.NULL_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.NUMBER_OBJECT_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.NUMBER_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.OBJECT_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.STRING_OBJECT_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.STRING_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.UNKNOWN_TYPE;
import static com.google.javascript.rhino.jstype.JSTypeNative.VOID_TYPE;
import com.google.common.base.Joiner;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.Maps;
import com.google.javascript.jscomp.CodingConvention.AssertionFunctionSpec;
import com.google.javascript.jscomp.CompilerOptions.LanguageMode;
import com.google.javascript.jscomp.DataFlowAnalysis.BranchedFlowState;
import com.google.javascript.jscomp.type.FlowScope;
import com.google.javascript.jscomp.type.ReverseAbstractInterpreter;
import com.google.javascript.jscomp.type.SemanticReverseAbstractInterpreter;
import com.google.javascript.rhino.Node;
import com.google.javascript.rhino.jstype.EnumType;
import com.google.javascript.rhino.jstype.JSType;
import com.google.javascript.rhino.jstype.JSTypeNative;
import com.google.javascript.rhino.jstype.JSTypeRegistry;
import com.google.javascript.rhino.jstype.StaticSlot;
import com.google.javascript.rhino.testing.Asserts;
import junit.framework.TestCase;
import java.util.Map;
/**
* Tests {@link TypeInference}.
*
*/
public class TypeInferenceTest extends TestCase {
private Compiler compiler;
private JSTypeRegistry registry;
private Map<String,JSType> assumptions;
private FlowScope returnScope;
private static final Map<String, AssertionFunctionSpec>
ASSERTION_FUNCTION_MAP = Maps.newHashMap();
static {
for (AssertionFunctionSpec func :
new ClosureCodingConvention().getAssertionFunctions()) {
ASSERTION_FUNCTION_MAP.put(func.getFunctionName(), func);
}
}
@Override
public void setUp() {
compiler = new Compiler();
CompilerOptions options = new CompilerOptions();
options.setLanguageIn(LanguageMode.ECMASCRIPT5);
compiler.initOptions(options);
registry = compiler.getTypeRegistry();
assumptions = Maps.newHashMap();
returnScope = null;
}
private void assuming(String name, JSType type) {
assumptions.put(name, type);
}
private void assuming(String name, JSTypeNative type) {
assuming(name, registry.getNativeType(type));
}
private void inFunction(String js) {
// Parse the body of the function.
Node root = compiler.parseTestCode("(function() {" + js + "});");
assertEquals("parsing error: " +
Joiner.on(", ").join(compiler.getErrors()),
0, compiler.getErrorCount());
Node n = root.getFirstChild().getFirstChild();
// Create the scope with the assumptions.
TypedScopeCreator scopeCreator = new TypedScopeCreator(compiler);
Scope assumedScope = scopeCreator.createScope(
n, scopeCreator.createScope(root, null));
for (Map.Entry<String,JSType> entry : assumptions.entrySet()) {
assumedScope.declare(entry.getKey(), null, entry.getValue(), null);
}
// Create the control graph.
ControlFlowAnalysis cfa = new ControlFlowAnalysis(compiler, false, false);
cfa.process(null, n);
ControlFlowGraph<Node> cfg = cfa.getCfg();
// Create a simple reverse abstract interpreter.
ReverseAbstractInterpreter rai = new SemanticReverseAbstractInterpreter(
compiler.getCodingConvention(), registry);
// Do the type inference by data-flow analysis.
TypeInference dfa = new TypeInference(compiler, cfg, rai, assumedScope,
ASSERTION_FUNCTION_MAP);
dfa.analyze();
// Get the scope of the implicit return.
BranchedFlowState<FlowScope> rtnState =
cfg.getImplicitReturn().getAnnotation();
returnScope = rtnState.getIn();
}
private JSType getType(String name) {
assertTrue("The return scope should not be null.", returnScope != null);
StaticSlot<JSType> var = returnScope.getSlot(name);
assertTrue("The variable " + name + " is missing from the scope.",
var != null);
return var.getType();
}
private void verify(String name, JSType type) {
Asserts.assertTypeEquals(type, getType(name));
}
private void verify(String name, JSTypeNative type) {
verify(name, registry.getNativeType(type));
}
private void verifySubtypeOf(String name, JSType type) {
JSType varType = getType(name);
assertTrue("The variable " + name + " is missing a type.", varType != null);
assertTrue("The type " + varType + " of variable " + name +
" is not a subtype of " + type +".", varType.isSubtype(type));
}
private void verifySubtypeOf(String name, JSTypeNative type) {
verifySubtypeOf(name, registry.getNativeType(type));
}
private EnumType createEnumType(String name, JSTypeNative elemType) {
return createEnumType(name, registry.getNativeType(elemType));
}
private EnumType createEnumType(String name, JSType elemType) {
return registry.createEnumType(name, null, elemType);
}
private JSType createUndefinableType(JSTypeNative type) {
return registry.createUnionType(
registry.getNativeType(type), registry.getNativeType(VOID_TYPE));
}
private JSType createNullableType(JSTypeNative type) {
return createNullableType(registry.getNativeType(type));
}
private JSType createNullableType(JSType type) {
return registry.createNullableType(type);
}
private JSType createUnionType(JSTypeNative type1, JSTypeNative type2) {
return registry.createUnionType(
registry.getNativeType(type1), registry.getNativeType(type2));
}
public void testAssumption() {
assuming("x", NUMBER_TYPE);
inFunction("");
verify("x", NUMBER_TYPE);
}
public void testVar() {
inFunction("var x = 1;");
verify("x", NUMBER_TYPE);
}
public void testEmptyVar() {
inFunction("var x;");
verify("x", VOID_TYPE);
}
public void testAssignment() {
assuming("x", OBJECT_TYPE);
inFunction("x = 1;");
verify("x", NUMBER_TYPE);
}
public void testGetProp() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("x.y();");
verify("x", OBJECT_TYPE);
}
public void testGetElemDereference() {
assuming("x", createUndefinableType(OBJECT_TYPE));
inFunction("x['z'] = 3;");
verify("x", OBJECT_TYPE);
}
public void testIf1() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = {}; if (x) { y = x; }");
verifySubtypeOf("y", OBJECT_TYPE);
}
public void testIf1a() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = {}; if (x != null) { y = x; }");
verifySubtypeOf("y", OBJECT_TYPE);
}
public void testIf2() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = x; if (x) { y = x; } else { y = {}; }");
verifySubtypeOf("y", OBJECT_TYPE);
}
public void testIf3() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = 1; if (x) { y = x; }");
verify("y", createUnionType(OBJECT_TYPE, NUMBER_TYPE));
}
public void testAssert1() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assert(x); out2 = x;");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
}
public void testAssert1a() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assert(x !== null); out2 = x;");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
}
public void testAssert2() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
inFunction("goog.asserts.assert(1, x); out1 = x;");
verify("out1", startType);
}
public void testAssert3() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
assuming("y", startType);
inFunction("out1 = x; goog.asserts.assert(x && y); out2 = x; out3 = y;");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
verify("out3", OBJECT_TYPE);
}
public void testAssert4() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
assuming("y", startType);
inFunction("out1 = x; goog.asserts.assert(x && !y); out2 = x; out3 = y;");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
verify("out3", NULL_TYPE);
}
public void testAssert5() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
assuming("y", startType);
inFunction("goog.asserts.assert(x || y); out1 = x; out2 = y;");
verify("out1", startType);
verify("out2", startType);
}
public void testAssert6() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x.y", startType);
inFunction("out1 = x.y; goog.asserts.assert(x.y); out2 = x.y;");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
}
public void testAssert7() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
inFunction("out1 = x; out2 = goog.asserts.assert(x);");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
}
public void testAssert8() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
inFunction("out1 = x; out2 = goog.asserts.assert(x != null);");
verify("out1", startType);
verify("out2", BOOLEAN_TYPE);
}
public void testAssert9() {
JSType startType = createNullableType(NUMBER_TYPE);
assuming("x", startType);
inFunction("out1 = x; out2 = goog.asserts.assert(y = x);");
verify("out1", startType);
verify("out2", NUMBER_TYPE);
}
public void testAssert10() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x", startType);
assuming("y", startType);
inFunction("out1 = x; out2 = goog.asserts.assert(x && y); out3 = x;");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
verify("out3", OBJECT_TYPE);
}
public void testAssertNumber() {
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertNumber(x); out2 = x;");
verify("out1", startType);
verify("out2", NUMBER_TYPE);
}
public void testAssertNumber2() {
// Make sure it ignores expressions.
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("goog.asserts.assertNumber(x + x); out1 = x;");
verify("out1", startType);
}
public void testAssertNumber3() {
// Make sure it ignores expressions.
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("out1 = x; out2 = goog.asserts.assertNumber(x + x);");
verify("out1", startType);
verify("out2", NUMBER_TYPE);
}
public void testAssertString() {
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertString(x); out2 = x;");
verify("out1", startType);
verify("out2", STRING_TYPE);
}
public void testAssertFunction() {
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertFunction(x); out2 = x;");
verify("out1", startType);
verifySubtypeOf("out2", FUNCTION_INSTANCE_TYPE);
}
public void testAssertObject() {
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertObject(x); out2 = x;");
verify("out1", startType);
verifySubtypeOf("out2", OBJECT_TYPE);
}
public void testAssertObject2() {
JSType startType = createNullableType(ARRAY_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertObject(x); out2 = x;");
verify("out1", startType);
verify("out2", ARRAY_TYPE);
}
public void testAssertObject3() {
JSType startType = createNullableType(OBJECT_TYPE);
assuming("x.y", startType);
inFunction("out1 = x.y; goog.asserts.assertObject(x.y); out2 = x.y;");
verify("out1", startType);
verify("out2", OBJECT_TYPE);
}
public void testAssertObject4() {
JSType startType = createNullableType(ARRAY_TYPE);
assuming("x", startType);
inFunction("out1 = x; out2 = goog.asserts.assertObject(x);");
verify("out1", startType);
verify("out2", ARRAY_TYPE);
}
public void testAssertObject5() {
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction(
"out1 = x;" +
"out2 = /** @type {!Array} */ (goog.asserts.assertObject(x));");
verify("out1", startType);
verify("out2", ARRAY_TYPE);
}
public void testAssertArray() {
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertArray(x); out2 = x;");
verify("out1", startType);
verifySubtypeOf("out2", ARRAY_TYPE);
}
public void testAssertInstanceof() {
JSType startType = createNullableType(ALL_TYPE);
assuming("x", startType);
inFunction("out1 = x; goog.asserts.assertInstanceof(x); out2 = x;");
verify("out1", startType);
verifySubtypeOf("out2", OBJECT_TYPE);
}
public void testReturn1() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("if (x) { return x; }\nx = {};\nreturn x;");
verify("x", OBJECT_TYPE);
}
public void testReturn2() {
assuming("x", createNullableType(NUMBER_TYPE));
inFunction("if (!x) { x = 0; }\nreturn x;");
verify("x", NUMBER_TYPE);
}
public void testWhile1() {
assuming("x", createNullableType(NUMBER_TYPE));
inFunction("while (!x) { if (x == null) { x = 0; } else { x = 1; } }");
verify("x", NUMBER_TYPE);
}
public void testWhile2() {
assuming("x", createNullableType(NUMBER_TYPE));
inFunction("while (!x) { x = {}; }");
verifySubtypeOf("x", createUnionType(OBJECT_TYPE, NUMBER_TYPE));
}
public void testDo() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("do { x = 1; } while (!x);");
verify("x", NUMBER_TYPE);
}
public void testFor1() {
assuming("y", NUMBER_TYPE);
inFunction("var x = null; var i = null; for (i=y; !i; i=1) { x = 1; }");
verify("x", createNullableType(NUMBER_TYPE));
verify("i", NUMBER_TYPE);
}
public void testFor2() {
assuming("y", OBJECT_TYPE);
inFunction("var x = null; var i = null; for (i in y) { x = 1; }");
verify("x", createNullableType(NUMBER_TYPE));
verify("i", createNullableType(STRING_TYPE));
}
public void testFor3() {
assuming("y", OBJECT_TYPE);
inFunction("var x = null; var i = null; for (var i in y) { x = 1; }");
verify("x", createNullableType(NUMBER_TYPE));
verify("i", createNullableType(STRING_TYPE));
}
public void testFor4() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = {};\n" +
"if (x) { for (var i = 0; i < 10; i++) { break; } y = x; }");
verifySubtypeOf("y", OBJECT_TYPE);
}
public void testSwitch1() {
assuming("x", NUMBER_TYPE);
inFunction("var y = null; switch(x) {\n" +
"case 1: y = 1; break;\n" +
"case 2: y = {};\n" +
"case 3: y = {};\n" +
"default: y = 0;}");
verify("y", NUMBER_TYPE);
}
public void testSwitch2() {
assuming("x", ALL_TYPE);
inFunction("var y = null; switch (typeof x) {\n" +
"case 'string':\n" +
" y = x;\n" +
" return;" +
"default:\n" +
" y = 'a';\n" +
"}");
verify("y", STRING_TYPE);
}
public void testSwitch3() {
assuming("x",
createNullableType(createUnionType(NUMBER_TYPE, STRING_TYPE)));
inFunction("var y; var z; switch (typeof x) {\n" +
"case 'string':\n" +
" y = 1; z = null;\n" +
" return;\n" +
"case 'number':\n" +
" y = x; z = null;\n" +
" return;" +
"default:\n" +
" y = 1; z = x;\n" +
"}");
verify("y", NUMBER_TYPE);
verify("z", NULL_TYPE);
}
public void testSwitch4() {
assuming("x", ALL_TYPE);
inFunction("var y = null; switch (typeof x) {\n" +
"case 'string':\n" +
"case 'number':\n" +
" y = x;\n" +
" return;\n" +
"default:\n" +
" y = 1;\n" +
"}\n");
verify("y", createUnionType(NUMBER_TYPE, STRING_TYPE));
}
public void testCall1() {
assuming("x",
createNullableType(
registry.createFunctionType(registry.getNativeType(NUMBER_TYPE))));
inFunction("var y = x();");
verify("y", NUMBER_TYPE);
}
public void testNew1() {
assuming("x",
createNullableType(
registry.getNativeType(JSTypeNative.U2U_CONSTRUCTOR_TYPE)));
inFunction("var y = new x();");
verify("y", JSTypeNative.NO_OBJECT_TYPE);
}
public void testInnerFunction1() {
inFunction("var x = 1; function f() { x = null; };");
verify("x", NUMBER_TYPE);
}
public void testInnerFunction2() {
inFunction("var x = 1; var f = function() { x = null; };");
verify("x", NUMBER_TYPE);
}
public void testHook() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = x ? x : {};");
verifySubtypeOf("y", OBJECT_TYPE);
}
public void testThrow() {
assuming("x", createNullableType(NUMBER_TYPE));
inFunction("var y = 1;\n" +
"if (x == null) { throw new Error('x is null') }\n" +
"y = x;");
verify("y", NUMBER_TYPE);
}
public void testTry1() {
assuming("x", NUMBER_TYPE);
inFunction("var y = null; try { y = null; } finally { y = x; }");
verify("y", NUMBER_TYPE);
}
public void testTry2() {
assuming("x", NUMBER_TYPE);
inFunction("var y = null;\n" +
"try { } catch (e) { y = null; } finally { y = x; }");
verify("y", NUMBER_TYPE);
}
public void testTry3() {
assuming("x", NUMBER_TYPE);
inFunction("var y = null; try { y = x; } catch (e) { }");
verify("y", NUMBER_TYPE);
}
public void testCatch1() {
inFunction("var y = null; try { foo(); } catch (e) { y = e; }");
verify("y", UNKNOWN_TYPE);
}
public void testCatch2() {
inFunction("var y = null; var e = 3; try { foo(); } catch (e) { y = e; }");
verify("y", UNKNOWN_TYPE);
}
public void testUnknownType1() {
inFunction("var y = 3; y = x;");
verify("y", UNKNOWN_TYPE);
}
public void testUnknownType2() {
assuming("x", ARRAY_TYPE);
inFunction("var y = 5; y = x[0];");
verify("y", UNKNOWN_TYPE);
}
public void testInfiniteLoop1() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("x = {}; while(x != null) { x = {}; }");
}
public void testInfiniteLoop2() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("x = {}; do { x = null; } while (x == null);");
}
public void testJoin1() {
JSType unknownOrNull = createUnionType(NULL_TYPE, UNKNOWN_TYPE);
assuming("x", BOOLEAN_TYPE);
assuming("unknownOrNull", unknownOrNull);
inFunction("var y; if (x) y = unknownOrNull; else y = null;");
verify("y", unknownOrNull);
}
public void testJoin2() {
JSType unknownOrNull = createUnionType(NULL_TYPE, UNKNOWN_TYPE);
assuming("x", BOOLEAN_TYPE);
assuming("unknownOrNull", unknownOrNull);
inFunction("var y; if (x) y = null; else y = unknownOrNull;");
verify("y", unknownOrNull);
}
public void testArrayLit() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = 3; if (x) { x = [y = x]; }");
verify("x", createUnionType(NULL_TYPE, ARRAY_TYPE));
verify("y", createUnionType(NUMBER_TYPE, OBJECT_TYPE));
}
public void testGetElem() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = 3; if (x) { x = x[y = x]; }");
verify("x", UNKNOWN_TYPE);
verify("y", createUnionType(NUMBER_TYPE, OBJECT_TYPE));
}
public void testEnumRAI1() {
JSType enumType = createEnumType("MyEnum", ARRAY_TYPE).getElementsType();
assuming("x", enumType);
inFunction("var y = null; if (x) y = x;");
verify("y", createNullableType(enumType));
}
public void testEnumRAI2() {
JSType enumType = createEnumType("MyEnum", NUMBER_TYPE).getElementsType();
assuming("x", enumType);
inFunction("var y = null; if (typeof x == 'number') y = x;");
verify("y", createNullableType(enumType));
}
public void testEnumRAI3() {
JSType enumType = createEnumType("MyEnum", NUMBER_TYPE).getElementsType();
assuming("x", enumType);
inFunction("var y = null; if (x && typeof x == 'number') y = x;");
verify("y", createNullableType(enumType));
}
public void testEnumRAI4() {
JSType enumType = createEnumType("MyEnum",
createUnionType(STRING_TYPE, NUMBER_TYPE)).getElementsType();
assuming("x", enumType);
inFunction("var y = null; if (typeof x == 'number') y = x;");
verify("y", createNullableType(NUMBER_TYPE));
}
public void testShortCircuitingAnd() {
assuming("x", NUMBER_TYPE);
inFunction("var y = null; if (x && (y = 3)) { }");
verify("y", createNullableType(NUMBER_TYPE));
}
public void testShortCircuitingAnd2() {
assuming("x", NUMBER_TYPE);
inFunction("var y = null; var z = 4; if (x && (y = 3)) { z = y; }");
verify("z", NUMBER_TYPE);
}
public void testShortCircuitingOr() {
assuming("x", NUMBER_TYPE);
inFunction("var y = null; if (x || (y = 3)) { }");
verify("y", createNullableType(NUMBER_TYPE));
}
public void testShortCircuitingOr2() {
assuming("x", NUMBER_TYPE);
inFunction("var y = null; var z = 4; if (x || (y = 3)) { z = y; }");
verify("z", createNullableType(NUMBER_TYPE));
}
public void testAssignInCondition() {
assuming("x", createNullableType(NUMBER_TYPE));
inFunction("var y; if (!(y = x)) { y = 3; }");
verify("y", NUMBER_TYPE);
}
public void testInstanceOf1() {
assuming("x", OBJECT_TYPE);
inFunction("var y = null; if (x instanceof String) y = x;");
verify("y", createNullableType(STRING_OBJECT_TYPE));
}
public void testInstanceOf2() {
assuming("x", createNullableType(OBJECT_TYPE));
inFunction("var y = 1; if (x instanceof String) y = x;");
verify("y", createUnionType(STRING_OBJECT_TYPE, NUMBER_TYPE));
}
public void testInstanceOf3() {
assuming("x", createUnionType(STRING_OBJECT_TYPE, NUMBER_OBJECT_TYPE));
inFunction("var y = null; if (x instanceof String) y = x;");
verify("y", createNullableType(STRING_OBJECT_TYPE));
}
public void testInstanceOf4() {
assuming("x", createUnionType(STRING_OBJECT_TYPE, NUMBER_OBJECT_TYPE));
inFunction("var y = null; if (x instanceof String); else y = x;");
verify("y", createNullableType(NUMBER_OBJECT_TYPE));
}
public void testInstanceOf5() {
assuming("x", OBJECT_TYPE);
inFunction("var y = null; if (x instanceof String); else y = x;");
verify("y", createNullableType(OBJECT_TYPE));
}
public void testFlattening() {
for (int i = 0; i < LinkedFlowScope.MAX_DEPTH + 1; i++) {
assuming("s" + i, ALL_TYPE);
}
assuming("b", JSTypeNative.BOOLEAN_TYPE);
StringBuilder body = new StringBuilder();
body.append("if (b) {");
for (int i = 0; i < LinkedFlowScope.MAX_DEPTH + 1; i++) {
body.append("s");
body.append(i);
body.append(" = 1;\n");
}
body.append(" } else { ");
for (int i = 0; i < LinkedFlowScope.MAX_DEPTH + 1; i++) {
body.append("s");
body.append(i);
body.append(" = 'ONE';\n");
}
body.append("}");
JSType numberORString = createUnionType(NUMBER_TYPE, STRING_TYPE);
inFunction(body.toString());
for (int i = 0; i < LinkedFlowScope.MAX_DEPTH + 1; i++) {
verify("s" + i, numberORString);
}
}
public void testUnary() {
assuming("x", NUMBER_TYPE);
inFunction("var y = +x;");
verify("y", NUMBER_TYPE);
inFunction("var z = -x;");
verify("z", NUMBER_TYPE);
}
public void testAdd1() {
assuming("x", NUMBER_TYPE);
inFunction("var y = x + 5;");
verify("y", NUMBER_TYPE);
}
public void testAdd2() {
assuming("x", NUMBER_TYPE);
inFunction("var y = x + '5';");
verify("y", STRING_TYPE);
}
public void testAdd3() {
assuming("x", NUMBER_TYPE);
inFunction("var y = '5' + x;");
verify("y", STRING_TYPE);
}
public void testAssignAdd() {
assuming("x", NUMBER_TYPE);
inFunction("x += '5';");
verify("x", STRING_TYPE);
}
public void testComparison() {
inFunction("var x = 'foo'; var y = (x = 3) < 4;");
verify("x", NUMBER_TYPE);
inFunction("var x = 'foo'; var y = (x = 3) > 4;");
verify("x", NUMBER_TYPE);
inFunction("var x = 'foo'; var y = (x = 3) <= 4;");
verify("x", NUMBER_TYPE);
inFunction("var x = 'foo'; var y = (x = 3) >= 4;");
verify("x", NUMBER_TYPE);
}
public void testThrownExpression() {
inFunction("var x = 'foo'; "
+ "try { throw new Error(x = 3); } catch (ex) {}");
verify("x", NUMBER_TYPE);
}
public void testObjectLit() {
inFunction("var x = {}; var out = x.a;");
verify("out", UNKNOWN_TYPE); // Shouldn't this be 'undefined'?
inFunction("var x = {a:1}; var out = x.a;");
verify("out", NUMBER_TYPE);
inFunction("var x = {a:1}; var out = x.a; x.a = 'string'; var out2 = x.a;");
verify("out", NUMBER_TYPE);
verify("out2", STRING_TYPE);
inFunction("var x = { get a() {return 1} }; var out = x.a;");
verify("out", UNKNOWN_TYPE);
inFunction(
"var x = {" +
" /** @return {number} */ get a() {return 1}" +
"};" +
"var out = x.a;");
verify("out", NUMBER_TYPE);
inFunction("var x = { set a(b) {} }; var out = x.a;");
verify("out", UNKNOWN_TYPE);
inFunction("var x = { " +
"/** @param {number} b */ set a(b) {} };" +
"var out = x.a;");
verify("out", NUMBER_TYPE);
}
public void testCast1() {
inFunction("var x = /** @type {Object} */ (this);");
verify("x", createNullableType(OBJECT_TYPE));
}
public void testCast2() {
inFunction(
"/** @return {boolean} */" +
"Object.prototype.method = function() { return true; };" +
"var x = /** @type {Object} */ (this).method;");
verify(
"x",
registry.createFunctionType(
registry.getNativeObjectType(OBJECT_TYPE),
registry.getNativeType(BOOLEAN_TYPE),
ImmutableList.<JSType>of() /* params */));
}
public void testBackwardsInferenceCall() {
inFunction(
"/** @param {{foo: (number|undefined)}} x */" +
"function f(x) {}" +
"var y = {};" +
"f(y);");
assertEquals("{foo: (number|undefined)}", getType("y").toString());
}
public void testBackwardsInferenceNew() {
inFunction(
"/**\n" +
" * @constructor\n" +
" * @param {{foo: (number|undefined)}} x\n" +
" */" +
"function F(x) {}" +
"var y = {};" +
"new F(y);");
assertEquals("{foo: (number|undefined)}", getType("y").toString());
}
} |
||
public void testParseOctal() throws Exception{
long value;
byte [] buffer;
final long MAX_OCTAL = 077777777777L; // Allowed 11 digits
final long MAX_OCTAL_OVERFLOW = 0777777777777L; // in fact 12 for some implementations
final String maxOctal = "777777777777"; // Maximum valid octal
buffer = maxOctal.getBytes(CharsetNames.UTF_8);
value = TarUtils.parseOctal(buffer,0, buffer.length);
assertEquals(MAX_OCTAL_OVERFLOW, value);
buffer[buffer.length - 1] = ' ';
value = TarUtils.parseOctal(buffer,0, buffer.length);
assertEquals(MAX_OCTAL, value);
buffer[buffer.length-1]=0;
value = TarUtils.parseOctal(buffer,0, buffer.length);
assertEquals(MAX_OCTAL, value);
buffer=new byte[]{0,0};
value = TarUtils.parseOctal(buffer,0, buffer.length);
assertEquals(0, value);
buffer=new byte[]{0,' '};
value = TarUtils.parseOctal(buffer,0, buffer.length);
assertEquals(0, value);
} | org.apache.commons.compress.archivers.tar.TarUtilsTest::testParseOctal | src/test/java/org/apache/commons/compress/archivers/tar/TarUtilsTest.java | 66 | src/test/java/org/apache/commons/compress/archivers/tar/TarUtilsTest.java | testParseOctal | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package org.apache.commons.compress.archivers.tar;
import junit.framework.TestCase;
import org.apache.commons.compress.archivers.zip.ZipEncoding;
import org.apache.commons.compress.archivers.zip.ZipEncodingHelper;
import org.apache.commons.compress.utils.CharsetNames;
public class TarUtilsTest extends TestCase {
public void testName(){
byte [] buff = new byte[20];
String sb1 = "abcdefghijklmnopqrstuvwxyz";
int off = TarUtils.formatNameBytes(sb1, buff, 1, buff.length-1);
assertEquals(off, 20);
String sb2 = TarUtils.parseName(buff, 1, 10);
assertEquals(sb2,sb1.substring(0,10));
sb2 = TarUtils.parseName(buff, 1, 19);
assertEquals(sb2,sb1.substring(0,19));
buff = new byte[30];
off = TarUtils.formatNameBytes(sb1, buff, 1, buff.length-1);
assertEquals(off, 30);
sb2 = TarUtils.parseName(buff, 1, buff.length-1);
assertEquals(sb1, sb2);
}
public void testParseOctal() throws Exception{
long value;
byte [] buffer;
final long MAX_OCTAL = 077777777777L; // Allowed 11 digits
final long MAX_OCTAL_OVERFLOW = 0777777777777L; // in fact 12 for some implementations
final String maxOctal = "777777777777"; // Maximum valid octal
buffer = maxOctal.getBytes(CharsetNames.UTF_8);
value = TarUtils.parseOctal(buffer,0, buffer.length);
assertEquals(MAX_OCTAL_OVERFLOW, value);
buffer[buffer.length - 1] = ' ';
value = TarUtils.parseOctal(buffer,0, buffer.length);
assertEquals(MAX_OCTAL, value);
buffer[buffer.length-1]=0;
value = TarUtils.parseOctal(buffer,0, buffer.length);
assertEquals(MAX_OCTAL, value);
buffer=new byte[]{0,0};
value = TarUtils.parseOctal(buffer,0, buffer.length);
assertEquals(0, value);
buffer=new byte[]{0,' '};
value = TarUtils.parseOctal(buffer,0, buffer.length);
assertEquals(0, value);
}
public void testParseOctalInvalid() throws Exception{
byte [] buffer;
buffer=new byte[0]; // empty byte array
try {
TarUtils.parseOctal(buffer,0, buffer.length);
fail("Expected IllegalArgumentException - should be at least 2 bytes long");
} catch (IllegalArgumentException expected) {
}
buffer=new byte[]{0}; // 1-byte array
try {
TarUtils.parseOctal(buffer,0, buffer.length);
fail("Expected IllegalArgumentException - should be at least 2 bytes long");
} catch (IllegalArgumentException expected) {
}
buffer=new byte[]{' ',0,0,0}; // not all NULs
try {
TarUtils.parseOctal(buffer,0, buffer.length);
fail("Expected IllegalArgumentException - not all NULs");
} catch (IllegalArgumentException expected) {
}
buffer = "abcdef ".getBytes(CharsetNames.UTF_8); // Invalid input
try {
TarUtils.parseOctal(buffer,0, buffer.length);
fail("Expected IllegalArgumentException");
} catch (IllegalArgumentException expected) {
}
buffer = " 0 07 ".getBytes(CharsetNames.UTF_8); // Invalid - embedded space
try {
TarUtils.parseOctal(buffer,0, buffer.length);
fail("Expected IllegalArgumentException - embedded space");
} catch (IllegalArgumentException expected) {
}
buffer = " 0\00007 ".getBytes(CharsetNames.UTF_8); // Invalid - embedded NUL
try {
TarUtils.parseOctal(buffer,0, buffer.length);
fail("Expected IllegalArgumentException - embedded NUL");
} catch (IllegalArgumentException expected) {
}
}
private void checkRoundTripOctal(final long value, final int bufsize) {
byte [] buffer = new byte[bufsize];
long parseValue;
TarUtils.formatLongOctalBytes(value, buffer, 0, buffer.length);
parseValue = TarUtils.parseOctal(buffer,0, buffer.length);
assertEquals(value,parseValue);
}
private void checkRoundTripOctal(final long value) {
checkRoundTripOctal(value, TarConstants.SIZELEN);
}
public void testRoundTripOctal() {
checkRoundTripOctal(0);
checkRoundTripOctal(1);
// checkRoundTripOctal(-1); // TODO What should this do?
checkRoundTripOctal(TarConstants.MAXSIZE);
// checkRoundTripOctal(0100000000000L); // TODO What should this do?
checkRoundTripOctal(0, TarConstants.UIDLEN);
checkRoundTripOctal(1, TarConstants.UIDLEN);
checkRoundTripOctal(TarConstants.MAXID, 8);
}
private void checkRoundTripOctalOrBinary(final long value, final int bufsize) {
byte [] buffer = new byte[bufsize];
long parseValue;
TarUtils.formatLongOctalOrBinaryBytes(value, buffer, 0, buffer.length);
parseValue = TarUtils.parseOctalOrBinary(buffer,0, buffer.length);
assertEquals(value,parseValue);
}
public void testRoundTripOctalOrBinary8() {
testRoundTripOctalOrBinary(8);
}
public void testRoundTripOctalOrBinary12() {
testRoundTripOctalOrBinary(12);
checkRoundTripOctalOrBinary(Long.MAX_VALUE, 12);
checkRoundTripOctalOrBinary(Long.MIN_VALUE + 1, 12);
}
private void testRoundTripOctalOrBinary(int length) {
checkRoundTripOctalOrBinary(0, length);
checkRoundTripOctalOrBinary(1, length);
checkRoundTripOctalOrBinary(TarConstants.MAXSIZE, length); // will need binary format
checkRoundTripOctalOrBinary(-1, length); // will need binary format
checkRoundTripOctalOrBinary(0xff00000000000001l, length);
}
// Check correct trailing bytes are generated
public void testTrailers() {
byte [] buffer = new byte[12];
TarUtils.formatLongOctalBytes(123, buffer, 0, buffer.length);
assertEquals(' ', buffer[buffer.length-1]);
assertEquals('3', buffer[buffer.length-2]); // end of number
TarUtils.formatOctalBytes(123, buffer, 0, buffer.length);
assertEquals(0 , buffer[buffer.length-1]);
assertEquals(' ', buffer[buffer.length-2]);
assertEquals('3', buffer[buffer.length-3]); // end of number
TarUtils.formatCheckSumOctalBytes(123, buffer, 0, buffer.length);
assertEquals(' ', buffer[buffer.length-1]);
assertEquals(0 , buffer[buffer.length-2]);
assertEquals('3', buffer[buffer.length-3]); // end of number
}
public void testNegative() throws Exception {
byte [] buffer = new byte[22];
TarUtils.formatUnsignedOctalString(-1, buffer, 0, buffer.length);
assertEquals("1777777777777777777777", new String(buffer, CharsetNames.UTF_8));
}
public void testOverflow() throws Exception {
byte [] buffer = new byte[8-1]; // a lot of the numbers have 8-byte buffers (nul term)
TarUtils.formatUnsignedOctalString(07777777L, buffer, 0, buffer.length);
assertEquals("7777777", new String(buffer, CharsetNames.UTF_8));
try {
TarUtils.formatUnsignedOctalString(017777777L, buffer, 0, buffer.length);
fail("Should have cause IllegalArgumentException");
} catch (IllegalArgumentException expected) {
}
}
public void testRoundTripNames(){
checkName("");
checkName("The quick brown fox\n");
checkName("\177");
// checkName("\0"); // does not work, because NUL is ignored
}
public void testRoundEncoding() throws Exception {
// COMPRESS-114
ZipEncoding enc = ZipEncodingHelper.getZipEncoding(CharsetNames.ISO_8859_1);
String s = "0302-0601-3\u00b1\u00b1\u00b1F06\u00b1W220\u00b1ZB\u00b1LALALA\u00b1\u00b1\u00b1\u00b1\u00b1\u00b1\u00b1\u00b1\u00b1\u00b1CAN\u00b1\u00b1DC\u00b1\u00b1\u00b104\u00b1060302\u00b1MOE.model";
byte buff[] = new byte[100];
int len = TarUtils.formatNameBytes(s, buff, 0, buff.length, enc);
assertEquals(s, TarUtils.parseName(buff, 0, len, enc));
}
private void checkName(String string) {
byte buff[] = new byte[100];
int len = TarUtils.formatNameBytes(string, buff, 0, buff.length);
assertEquals(string, TarUtils.parseName(buff, 0, len));
}
public void testReadNegativeBinary8Byte() {
byte[] b = new byte[] {
(byte) 0xff, (byte) 0xff, (byte) 0xff, (byte) 0xff,
(byte) 0xff, (byte) 0xff, (byte) 0xf1, (byte) 0xef,
};
assertEquals(-3601l, TarUtils.parseOctalOrBinary(b, 0, 8));
}
public void testReadNegativeBinary12Byte() {
byte[] b = new byte[] {
(byte) 0xff, (byte) 0xff, (byte) 0xff, (byte) 0xff,
(byte) 0xff, (byte) 0xff, (byte) 0xff, (byte) 0xff,
(byte) 0xff, (byte) 0xff, (byte) 0xf1, (byte) 0xef,
};
assertEquals(-3601l, TarUtils.parseOctalOrBinary(b, 0, 12));
}
public void testWriteNegativeBinary8Byte() {
byte[] b = new byte[] {
(byte) 0xff, (byte) 0xff, (byte) 0xff, (byte) 0xff,
(byte) 0xff, (byte) 0xff, (byte) 0xf1, (byte) 0xef,
};
assertEquals(-3601l, TarUtils.parseOctalOrBinary(b, 0, 8));
}
// https://issues.apache.org/jira/browse/COMPRESS-191
public void testVerifyHeaderCheckSum() {
byte[] valid = { // from bla.tar
116, 101, 115, 116, 49, 46, 120, 109, 108, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 48, 48, 48, 48, 54, 52, 52, 0, 48, 48, 48, 48, 55, 54, 53,
0, 48, 48, 48, 48, 55, 54, 53, 0, 48, 48, 48, 48, 48, 48, 48,
49, 49, 52, 50, 0, 49, 48, 55, 49, 54, 53, 52, 53, 54, 50, 54,
0, 48, 49, 50, 50, 54, 48, 0, 32, 48, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 117, 115, 116, 97, 114, 32, 32, 0,
116, 99, 117, 114, 100, 116, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 116, 99, 117,
114, 100, 116, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0 };
assertTrue(TarUtils.verifyCheckSum(valid));
byte[] compress117 = { // from COMPRESS-117
116, 101, 115, 116, 49, 46, 120, 109, 108, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 48, 48, 48, 48, 54, 52, 52, 0, 48, 48, 48, 48, 55, 54, 53,
0, 48, 48, 48, 48, 55, 54, 53, 0, 48, 48, 48, 48, 48, 48, 48,
49, 49, 52, 50, 0, 49, 48, 55, 49, 54, 53, 52, 53, 54, 50, 54,
0, 48, 49, 50, 50, 54, 48, 0, 32, 48, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 };
assertTrue(TarUtils.verifyCheckSum(compress117));
byte[] invalid = { // from the testAIFF.aif file in Tika
70, 79, 82, 77, 0, 0, 15, 46, 65, 73, 70, 70, 67, 79, 77, 77,
0, 0, 0, 18, 0, 2, 0, 0, 3, -64, 0, 16, 64, 14, -84, 68, 0, 0,
0, 0, 0, 0, 83, 83, 78, 68, 0, 0, 15, 8, 0, 0, 0, 0, 0, 0, 0,
0, 0, 1, -1, -1, 0, 1, -1, -1, 0, 0, 0, 0, 0, 0, 0, 1, -1, -1,
0, 0, 0, 0, 0, 0, -1, -1, 0, 2, -1, -2, 0, 2, -1, -1, 0, 0, 0,
0, 0, 0, 0, 0, 0, 1, -1, -1, 0, 0, -1, -1, 0, 1, 0, 0, 0, 0,
0, 0, 0, 1, -1, -1, 0, 1, -1, -2, 0, 1, -1, -1, 0, 1, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, -1, -1, 0,
2, -1, -2, 0, 2, -1, -1, 0, 0, 0, 1, -1, -1, 0, 1, -1, -1, 0,
0, 0, 0, 0, 0, 0, 0, 0, 1, -1, -2, 0, 2, -1, -2, 0, 1, 0, 0,
0, 1, -1, -1, 0, 0, 0, 1, -1, -1, 0, 0, 0, 1, -1, -2, 0, 2,
-1, -1, 0, 0, 0, 0, 0, 0, -1, -1, 0, 1, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, -1, -1, 0, 1, -1, -1, 0, 2, -1, -2,
0, 2, -1, -2, 0, 2, -1, -2, 0, 1, 0, 0, 0, 0, 0, 0, 0, 1, -1,
-2, 0, 2, -1, -2, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
-1, -1, 0, 1, 0, 0, -1, -1, 0, 2, -1, -2, 0, 2, -1, -1, 0, 0,
0, 0, 0, 0, -1, -1, 0, 1, -1, -1, 0, 1, -1, -1, 0, 2, -1, -2,
0, 1, 0, 0, -1, -1, 0, 2, -1, -2, 0, 2, -1, -2, 0, 1, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, -1, -1, 0, 0, 0,
0, -1, -1, 0, 1, 0, 0, 0, 0, 0, 1, -1, -1, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, -1, -2, 0, 2, -1, -1, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, -1, -2, 0, 1, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 1, -1, -1, 0, 0, 0, 0, -1, -1, 0, 2, -1, -2,
0, 2, -1, -2, 0, 2, -1, -1, 0, 0, 0, 0, -1, -1, 0, 1, -1, -1,
0, 1, -1, -1, 0, 1, -1, -1, 0, 1, -1, -1, 0, 1, 0, 0, 0, 0,
-1, -1, 0, 2, -1, -2, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
1, -1, -1, 0, 0, 0, 0, -1, -1, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 1 };
assertFalse(TarUtils.verifyCheckSum(invalid));
}
} | // You are a professional Java test case writer, please create a test case named `testParseOctal` for the issue `Compress-COMPRESS-262`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Compress-COMPRESS-262
//
// ## Issue-Title:
// TarArchiveInputStream fails to read entry with big user-id value
//
// ## Issue-Description:
//
// Caused by: java.lang.IllegalArgumentException: Invalid byte 52 at offset 7 in '62410554' len=8
//
// at org.apache.commons.compress.archivers.tar.TarUtils.parseOctal(TarUtils.java:130)
//
// at org.apache.commons.compress.archivers.tar.TarUtils.parseOctalOrBinary(TarUtils.java:175)
//
// at org.apache.commons.compress.archivers.tar.TarArchiveEntry.parseTarHeader(TarArchiveEntry.java:953)
//
// at org.apache.commons.compress.archivers.tar.TarArchiveEntry.parseTarHeader(TarArchiveEntry.java:940)
//
// at org.apache.commons.compress.archivers.tar.TarArchiveEntry.<init>(TarArchiveEntry.java:324)
//
// at org.apache.commons.compress.archivers.tar.TarArchiveInputStream.getNextTarEntry(TarArchiveInputStream.java:247)
//
// ... 5 more
//
//
//
//
//
public void testParseOctal() throws Exception {
| 66 | 24 | 45 | src/test/java/org/apache/commons/compress/archivers/tar/TarUtilsTest.java | src/test/java | ```markdown
## Issue-ID: Compress-COMPRESS-262
## Issue-Title:
TarArchiveInputStream fails to read entry with big user-id value
## Issue-Description:
Caused by: java.lang.IllegalArgumentException: Invalid byte 52 at offset 7 in '62410554' len=8
at org.apache.commons.compress.archivers.tar.TarUtils.parseOctal(TarUtils.java:130)
at org.apache.commons.compress.archivers.tar.TarUtils.parseOctalOrBinary(TarUtils.java:175)
at org.apache.commons.compress.archivers.tar.TarArchiveEntry.parseTarHeader(TarArchiveEntry.java:953)
at org.apache.commons.compress.archivers.tar.TarArchiveEntry.parseTarHeader(TarArchiveEntry.java:940)
at org.apache.commons.compress.archivers.tar.TarArchiveEntry.<init>(TarArchiveEntry.java:324)
at org.apache.commons.compress.archivers.tar.TarArchiveInputStream.getNextTarEntry(TarArchiveInputStream.java:247)
... 5 more
```
You are a professional Java test case writer, please create a test case named `testParseOctal` for the issue `Compress-COMPRESS-262`, utilizing the provided issue report information and the following function signature.
```java
public void testParseOctal() throws Exception {
```
| 45 | [
"org.apache.commons.compress.archivers.tar.TarUtils"
] | 93dc27dfb9a30dd541c5272638d9ca2d58177b88d4e0789c5f79f85d79fa3357 | public void testParseOctal() throws Exception | // You are a professional Java test case writer, please create a test case named `testParseOctal` for the issue `Compress-COMPRESS-262`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Compress-COMPRESS-262
//
// ## Issue-Title:
// TarArchiveInputStream fails to read entry with big user-id value
//
// ## Issue-Description:
//
// Caused by: java.lang.IllegalArgumentException: Invalid byte 52 at offset 7 in '62410554' len=8
//
// at org.apache.commons.compress.archivers.tar.TarUtils.parseOctal(TarUtils.java:130)
//
// at org.apache.commons.compress.archivers.tar.TarUtils.parseOctalOrBinary(TarUtils.java:175)
//
// at org.apache.commons.compress.archivers.tar.TarArchiveEntry.parseTarHeader(TarArchiveEntry.java:953)
//
// at org.apache.commons.compress.archivers.tar.TarArchiveEntry.parseTarHeader(TarArchiveEntry.java:940)
//
// at org.apache.commons.compress.archivers.tar.TarArchiveEntry.<init>(TarArchiveEntry.java:324)
//
// at org.apache.commons.compress.archivers.tar.TarArchiveInputStream.getNextTarEntry(TarArchiveInputStream.java:247)
//
// ... 5 more
//
//
//
//
//
| Compress | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package org.apache.commons.compress.archivers.tar;
import junit.framework.TestCase;
import org.apache.commons.compress.archivers.zip.ZipEncoding;
import org.apache.commons.compress.archivers.zip.ZipEncodingHelper;
import org.apache.commons.compress.utils.CharsetNames;
public class TarUtilsTest extends TestCase {
public void testName(){
byte [] buff = new byte[20];
String sb1 = "abcdefghijklmnopqrstuvwxyz";
int off = TarUtils.formatNameBytes(sb1, buff, 1, buff.length-1);
assertEquals(off, 20);
String sb2 = TarUtils.parseName(buff, 1, 10);
assertEquals(sb2,sb1.substring(0,10));
sb2 = TarUtils.parseName(buff, 1, 19);
assertEquals(sb2,sb1.substring(0,19));
buff = new byte[30];
off = TarUtils.formatNameBytes(sb1, buff, 1, buff.length-1);
assertEquals(off, 30);
sb2 = TarUtils.parseName(buff, 1, buff.length-1);
assertEquals(sb1, sb2);
}
public void testParseOctal() throws Exception{
long value;
byte [] buffer;
final long MAX_OCTAL = 077777777777L; // Allowed 11 digits
final long MAX_OCTAL_OVERFLOW = 0777777777777L; // in fact 12 for some implementations
final String maxOctal = "777777777777"; // Maximum valid octal
buffer = maxOctal.getBytes(CharsetNames.UTF_8);
value = TarUtils.parseOctal(buffer,0, buffer.length);
assertEquals(MAX_OCTAL_OVERFLOW, value);
buffer[buffer.length - 1] = ' ';
value = TarUtils.parseOctal(buffer,0, buffer.length);
assertEquals(MAX_OCTAL, value);
buffer[buffer.length-1]=0;
value = TarUtils.parseOctal(buffer,0, buffer.length);
assertEquals(MAX_OCTAL, value);
buffer=new byte[]{0,0};
value = TarUtils.parseOctal(buffer,0, buffer.length);
assertEquals(0, value);
buffer=new byte[]{0,' '};
value = TarUtils.parseOctal(buffer,0, buffer.length);
assertEquals(0, value);
}
public void testParseOctalInvalid() throws Exception{
byte [] buffer;
buffer=new byte[0]; // empty byte array
try {
TarUtils.parseOctal(buffer,0, buffer.length);
fail("Expected IllegalArgumentException - should be at least 2 bytes long");
} catch (IllegalArgumentException expected) {
}
buffer=new byte[]{0}; // 1-byte array
try {
TarUtils.parseOctal(buffer,0, buffer.length);
fail("Expected IllegalArgumentException - should be at least 2 bytes long");
} catch (IllegalArgumentException expected) {
}
buffer=new byte[]{' ',0,0,0}; // not all NULs
try {
TarUtils.parseOctal(buffer,0, buffer.length);
fail("Expected IllegalArgumentException - not all NULs");
} catch (IllegalArgumentException expected) {
}
buffer = "abcdef ".getBytes(CharsetNames.UTF_8); // Invalid input
try {
TarUtils.parseOctal(buffer,0, buffer.length);
fail("Expected IllegalArgumentException");
} catch (IllegalArgumentException expected) {
}
buffer = " 0 07 ".getBytes(CharsetNames.UTF_8); // Invalid - embedded space
try {
TarUtils.parseOctal(buffer,0, buffer.length);
fail("Expected IllegalArgumentException - embedded space");
} catch (IllegalArgumentException expected) {
}
buffer = " 0\00007 ".getBytes(CharsetNames.UTF_8); // Invalid - embedded NUL
try {
TarUtils.parseOctal(buffer,0, buffer.length);
fail("Expected IllegalArgumentException - embedded NUL");
} catch (IllegalArgumentException expected) {
}
}
private void checkRoundTripOctal(final long value, final int bufsize) {
byte [] buffer = new byte[bufsize];
long parseValue;
TarUtils.formatLongOctalBytes(value, buffer, 0, buffer.length);
parseValue = TarUtils.parseOctal(buffer,0, buffer.length);
assertEquals(value,parseValue);
}
private void checkRoundTripOctal(final long value) {
checkRoundTripOctal(value, TarConstants.SIZELEN);
}
public void testRoundTripOctal() {
checkRoundTripOctal(0);
checkRoundTripOctal(1);
// checkRoundTripOctal(-1); // TODO What should this do?
checkRoundTripOctal(TarConstants.MAXSIZE);
// checkRoundTripOctal(0100000000000L); // TODO What should this do?
checkRoundTripOctal(0, TarConstants.UIDLEN);
checkRoundTripOctal(1, TarConstants.UIDLEN);
checkRoundTripOctal(TarConstants.MAXID, 8);
}
private void checkRoundTripOctalOrBinary(final long value, final int bufsize) {
byte [] buffer = new byte[bufsize];
long parseValue;
TarUtils.formatLongOctalOrBinaryBytes(value, buffer, 0, buffer.length);
parseValue = TarUtils.parseOctalOrBinary(buffer,0, buffer.length);
assertEquals(value,parseValue);
}
public void testRoundTripOctalOrBinary8() {
testRoundTripOctalOrBinary(8);
}
public void testRoundTripOctalOrBinary12() {
testRoundTripOctalOrBinary(12);
checkRoundTripOctalOrBinary(Long.MAX_VALUE, 12);
checkRoundTripOctalOrBinary(Long.MIN_VALUE + 1, 12);
}
private void testRoundTripOctalOrBinary(int length) {
checkRoundTripOctalOrBinary(0, length);
checkRoundTripOctalOrBinary(1, length);
checkRoundTripOctalOrBinary(TarConstants.MAXSIZE, length); // will need binary format
checkRoundTripOctalOrBinary(-1, length); // will need binary format
checkRoundTripOctalOrBinary(0xff00000000000001l, length);
}
// Check correct trailing bytes are generated
public void testTrailers() {
byte [] buffer = new byte[12];
TarUtils.formatLongOctalBytes(123, buffer, 0, buffer.length);
assertEquals(' ', buffer[buffer.length-1]);
assertEquals('3', buffer[buffer.length-2]); // end of number
TarUtils.formatOctalBytes(123, buffer, 0, buffer.length);
assertEquals(0 , buffer[buffer.length-1]);
assertEquals(' ', buffer[buffer.length-2]);
assertEquals('3', buffer[buffer.length-3]); // end of number
TarUtils.formatCheckSumOctalBytes(123, buffer, 0, buffer.length);
assertEquals(' ', buffer[buffer.length-1]);
assertEquals(0 , buffer[buffer.length-2]);
assertEquals('3', buffer[buffer.length-3]); // end of number
}
public void testNegative() throws Exception {
byte [] buffer = new byte[22];
TarUtils.formatUnsignedOctalString(-1, buffer, 0, buffer.length);
assertEquals("1777777777777777777777", new String(buffer, CharsetNames.UTF_8));
}
public void testOverflow() throws Exception {
byte [] buffer = new byte[8-1]; // a lot of the numbers have 8-byte buffers (nul term)
TarUtils.formatUnsignedOctalString(07777777L, buffer, 0, buffer.length);
assertEquals("7777777", new String(buffer, CharsetNames.UTF_8));
try {
TarUtils.formatUnsignedOctalString(017777777L, buffer, 0, buffer.length);
fail("Should have cause IllegalArgumentException");
} catch (IllegalArgumentException expected) {
}
}
public void testRoundTripNames(){
checkName("");
checkName("The quick brown fox\n");
checkName("\177");
// checkName("\0"); // does not work, because NUL is ignored
}
public void testRoundEncoding() throws Exception {
// COMPRESS-114
ZipEncoding enc = ZipEncodingHelper.getZipEncoding(CharsetNames.ISO_8859_1);
String s = "0302-0601-3\u00b1\u00b1\u00b1F06\u00b1W220\u00b1ZB\u00b1LALALA\u00b1\u00b1\u00b1\u00b1\u00b1\u00b1\u00b1\u00b1\u00b1\u00b1CAN\u00b1\u00b1DC\u00b1\u00b1\u00b104\u00b1060302\u00b1MOE.model";
byte buff[] = new byte[100];
int len = TarUtils.formatNameBytes(s, buff, 0, buff.length, enc);
assertEquals(s, TarUtils.parseName(buff, 0, len, enc));
}
private void checkName(String string) {
byte buff[] = new byte[100];
int len = TarUtils.formatNameBytes(string, buff, 0, buff.length);
assertEquals(string, TarUtils.parseName(buff, 0, len));
}
public void testReadNegativeBinary8Byte() {
byte[] b = new byte[] {
(byte) 0xff, (byte) 0xff, (byte) 0xff, (byte) 0xff,
(byte) 0xff, (byte) 0xff, (byte) 0xf1, (byte) 0xef,
};
assertEquals(-3601l, TarUtils.parseOctalOrBinary(b, 0, 8));
}
public void testReadNegativeBinary12Byte() {
byte[] b = new byte[] {
(byte) 0xff, (byte) 0xff, (byte) 0xff, (byte) 0xff,
(byte) 0xff, (byte) 0xff, (byte) 0xff, (byte) 0xff,
(byte) 0xff, (byte) 0xff, (byte) 0xf1, (byte) 0xef,
};
assertEquals(-3601l, TarUtils.parseOctalOrBinary(b, 0, 12));
}
public void testWriteNegativeBinary8Byte() {
byte[] b = new byte[] {
(byte) 0xff, (byte) 0xff, (byte) 0xff, (byte) 0xff,
(byte) 0xff, (byte) 0xff, (byte) 0xf1, (byte) 0xef,
};
assertEquals(-3601l, TarUtils.parseOctalOrBinary(b, 0, 8));
}
// https://issues.apache.org/jira/browse/COMPRESS-191
public void testVerifyHeaderCheckSum() {
byte[] valid = { // from bla.tar
116, 101, 115, 116, 49, 46, 120, 109, 108, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 48, 48, 48, 48, 54, 52, 52, 0, 48, 48, 48, 48, 55, 54, 53,
0, 48, 48, 48, 48, 55, 54, 53, 0, 48, 48, 48, 48, 48, 48, 48,
49, 49, 52, 50, 0, 49, 48, 55, 49, 54, 53, 52, 53, 54, 50, 54,
0, 48, 49, 50, 50, 54, 48, 0, 32, 48, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 117, 115, 116, 97, 114, 32, 32, 0,
116, 99, 117, 114, 100, 116, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 116, 99, 117,
114, 100, 116, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0 };
assertTrue(TarUtils.verifyCheckSum(valid));
byte[] compress117 = { // from COMPRESS-117
116, 101, 115, 116, 49, 46, 120, 109, 108, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 48, 48, 48, 48, 54, 52, 52, 0, 48, 48, 48, 48, 55, 54, 53,
0, 48, 48, 48, 48, 55, 54, 53, 0, 48, 48, 48, 48, 48, 48, 48,
49, 49, 52, 50, 0, 49, 48, 55, 49, 54, 53, 52, 53, 54, 50, 54,
0, 48, 49, 50, 50, 54, 48, 0, 32, 48, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 };
assertTrue(TarUtils.verifyCheckSum(compress117));
byte[] invalid = { // from the testAIFF.aif file in Tika
70, 79, 82, 77, 0, 0, 15, 46, 65, 73, 70, 70, 67, 79, 77, 77,
0, 0, 0, 18, 0, 2, 0, 0, 3, -64, 0, 16, 64, 14, -84, 68, 0, 0,
0, 0, 0, 0, 83, 83, 78, 68, 0, 0, 15, 8, 0, 0, 0, 0, 0, 0, 0,
0, 0, 1, -1, -1, 0, 1, -1, -1, 0, 0, 0, 0, 0, 0, 0, 1, -1, -1,
0, 0, 0, 0, 0, 0, -1, -1, 0, 2, -1, -2, 0, 2, -1, -1, 0, 0, 0,
0, 0, 0, 0, 0, 0, 1, -1, -1, 0, 0, -1, -1, 0, 1, 0, 0, 0, 0,
0, 0, 0, 1, -1, -1, 0, 1, -1, -2, 0, 1, -1, -1, 0, 1, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, -1, -1, 0,
2, -1, -2, 0, 2, -1, -1, 0, 0, 0, 1, -1, -1, 0, 1, -1, -1, 0,
0, 0, 0, 0, 0, 0, 0, 0, 1, -1, -2, 0, 2, -1, -2, 0, 1, 0, 0,
0, 1, -1, -1, 0, 0, 0, 1, -1, -1, 0, 0, 0, 1, -1, -2, 0, 2,
-1, -1, 0, 0, 0, 0, 0, 0, -1, -1, 0, 1, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, -1, -1, 0, 1, -1, -1, 0, 2, -1, -2,
0, 2, -1, -2, 0, 2, -1, -2, 0, 1, 0, 0, 0, 0, 0, 0, 0, 1, -1,
-2, 0, 2, -1, -2, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
-1, -1, 0, 1, 0, 0, -1, -1, 0, 2, -1, -2, 0, 2, -1, -1, 0, 0,
0, 0, 0, 0, -1, -1, 0, 1, -1, -1, 0, 1, -1, -1, 0, 2, -1, -2,
0, 1, 0, 0, -1, -1, 0, 2, -1, -2, 0, 2, -1, -2, 0, 1, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, -1, -1, 0, 0, 0,
0, -1, -1, 0, 1, 0, 0, 0, 0, 0, 1, -1, -1, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, -1, -2, 0, 2, -1, -1, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, -1, -2, 0, 1, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 1, -1, -1, 0, 0, 0, 0, -1, -1, 0, 2, -1, -2,
0, 2, -1, -2, 0, 2, -1, -1, 0, 0, 0, 0, -1, -1, 0, 1, -1, -1,
0, 1, -1, -1, 0, 1, -1, -1, 0, 1, -1, -1, 0, 1, 0, 0, 0, 0,
-1, -1, 0, 2, -1, -2, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
1, -1, -1, 0, 0, 0, 0, -1, -1, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 1 };
assertFalse(TarUtils.verifyCheckSum(invalid));
}
} |
||
@Test
public void testLinearCombinationWithSingleElementArray() {
final double[] a = { 1.23456789 };
final double[] b = { 98765432.1 };
Assert.assertEquals(a[0] * b[0], MathArrays.linearCombination(a, b), 0d);
} | org.apache.commons.math3.util.MathArraysTest::testLinearCombinationWithSingleElementArray | src/test/java/org/apache/commons/math3/util/MathArraysTest.java | 591 | src/test/java/org/apache/commons/math3/util/MathArraysTest.java | testLinearCombinationWithSingleElementArray | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with this
* work for additional information regarding copyright ownership. The ASF
* licenses this file to You under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0 Unless required by applicable law
* or agreed to in writing, software distributed under the License is
* distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package org.apache.commons.math3.util;
import java.util.Arrays;
import org.apache.commons.math3.TestUtils;
import org.apache.commons.math3.exception.DimensionMismatchException;
import org.apache.commons.math3.exception.MathArithmeticException;
import org.apache.commons.math3.exception.MathIllegalArgumentException;
import org.apache.commons.math3.exception.NoDataException;
import org.apache.commons.math3.exception.NonMonotonicSequenceException;
import org.apache.commons.math3.exception.NotPositiveException;
import org.apache.commons.math3.exception.NotStrictlyPositiveException;
import org.apache.commons.math3.exception.NullArgumentException;
import org.apache.commons.math3.random.Well1024a;
import org.junit.Assert;
import org.junit.Test;
/**
* Test cases for the {@link MathArrays} class.
*
* @version $Id$
*/
public class MathArraysTest {
@Test
public void testScale() {
final double[] test = new double[] { -2.5, -1, 0, 1, 2.5 };
final double[] correctTest = MathArrays.copyOf(test);
final double[] correctScaled = new double[]{5.25, 2.1, 0, -2.1, -5.25};
final double[] scaled = MathArrays.scale(-2.1, test);
// Make sure test has not changed
for (int i = 0; i < test.length; i++) {
Assert.assertEquals(correctTest[i], test[i], 0);
}
// Test scaled values
for (int i = 0; i < scaled.length; i++) {
Assert.assertEquals(correctScaled[i], scaled[i], 0);
}
}
@Test
public void testScaleInPlace() {
final double[] test = new double[] { -2.5, -1, 0, 1, 2.5 };
final double[] correctScaled = new double[]{5.25, 2.1, 0, -2.1, -5.25};
MathArrays.scaleInPlace(-2.1, test);
// Make sure test has changed
for (int i = 0; i < test.length; i++) {
Assert.assertEquals(correctScaled[i], test[i], 0);
}
}
@Test(expected=DimensionMismatchException.class)
public void testEbeAddPrecondition() {
MathArrays.ebeAdd(new double[3], new double[4]);
}
@Test(expected=DimensionMismatchException.class)
public void testEbeSubtractPrecondition() {
MathArrays.ebeSubtract(new double[3], new double[4]);
}
@Test(expected=DimensionMismatchException.class)
public void testEbeMultiplyPrecondition() {
MathArrays.ebeMultiply(new double[3], new double[4]);
}
@Test(expected=DimensionMismatchException.class)
public void testEbeDividePrecondition() {
MathArrays.ebeDivide(new double[3], new double[4]);
}
@Test
public void testEbeAdd() {
final double[] a = { 0, 1, 2 };
final double[] b = { 3, 5, 7 };
final double[] r = MathArrays.ebeAdd(a, b);
for (int i = 0; i < a.length; i++) {
Assert.assertEquals(a[i] + b[i], r[i], 0);
}
}
@Test
public void testEbeSubtract() {
final double[] a = { 0, 1, 2 };
final double[] b = { 3, 5, 7 };
final double[] r = MathArrays.ebeSubtract(a, b);
for (int i = 0; i < a.length; i++) {
Assert.assertEquals(a[i] - b[i], r[i], 0);
}
}
@Test
public void testEbeMultiply() {
final double[] a = { 0, 1, 2 };
final double[] b = { 3, 5, 7 };
final double[] r = MathArrays.ebeMultiply(a, b);
for (int i = 0; i < a.length; i++) {
Assert.assertEquals(a[i] * b[i], r[i], 0);
}
}
@Test
public void testEbeDivide() {
final double[] a = { 0, 1, 2 };
final double[] b = { 3, 5, 7 };
final double[] r = MathArrays.ebeDivide(a, b);
for (int i = 0; i < a.length; i++) {
Assert.assertEquals(a[i] / b[i], r[i], 0);
}
}
@Test
public void testL1DistanceDouble() {
double[] p1 = { 2.5, 0.0 };
double[] p2 = { -0.5, 4.0 };
Assert.assertTrue(Precision.equals(7.0, MathArrays.distance1(p1, p2), 1));
}
@Test
public void testL1DistanceInt() {
int[] p1 = { 3, 0 };
int[] p2 = { 0, 4 };
Assert.assertEquals(7, MathArrays.distance1(p1, p2));
}
@Test
public void testL2DistanceDouble() {
double[] p1 = { 2.5, 0.0 };
double[] p2 = { -0.5, 4.0 };
Assert.assertTrue(Precision.equals(5.0, MathArrays.distance(p1, p2), 1));
}
@Test
public void testL2DistanceInt() {
int[] p1 = { 3, 0 };
int[] p2 = { 0, 4 };
Assert.assertTrue(Precision.equals(5, MathArrays.distance(p1, p2), 1));
}
@Test
public void testLInfDistanceDouble() {
double[] p1 = { 2.5, 0.0 };
double[] p2 = { -0.5, 4.0 };
Assert.assertTrue(Precision.equals(4.0, MathArrays.distanceInf(p1, p2), 1));
}
@Test
public void testLInfDistanceInt() {
int[] p1 = { 3, 0 };
int[] p2 = { 0, 4 };
Assert.assertEquals(4, MathArrays.distanceInf(p1, p2));
}
@Test
public void testCheckOrder() {
MathArrays.checkOrder(new double[] {-15, -5.5, -1, 2, 15},
MathArrays.OrderDirection.INCREASING, true);
MathArrays.checkOrder(new double[] {-15, -5.5, -1, 2, 2},
MathArrays.OrderDirection.INCREASING, false);
MathArrays.checkOrder(new double[] {3, -5.5, -11, -27.5},
MathArrays.OrderDirection.DECREASING, true);
MathArrays.checkOrder(new double[] {3, 0, 0, -5.5, -11, -27.5},
MathArrays.OrderDirection.DECREASING, false);
try {
MathArrays.checkOrder(new double[] {-15, -5.5, -1, -1, 2, 15},
MathArrays.OrderDirection.INCREASING, true);
Assert.fail("an exception should have been thrown");
} catch (NonMonotonicSequenceException e) {
// Expected
}
try {
MathArrays.checkOrder(new double[] {-15, -5.5, -1, -2, 2},
MathArrays.OrderDirection.INCREASING, false);
Assert.fail("an exception should have been thrown");
} catch (NonMonotonicSequenceException e) {
// Expected
}
try {
MathArrays.checkOrder(new double[] {3, 3, -5.5, -11, -27.5},
MathArrays.OrderDirection.DECREASING, true);
Assert.fail("an exception should have been thrown");
} catch (NonMonotonicSequenceException e) {
// Expected
}
try {
MathArrays.checkOrder(new double[] {3, -1, 0, -5.5, -11, -27.5},
MathArrays.OrderDirection.DECREASING, false);
Assert.fail("an exception should have been thrown");
} catch (NonMonotonicSequenceException e) {
// Expected
}
try {
MathArrays.checkOrder(new double[] {3, 0, -5.5, -11, -10},
MathArrays.OrderDirection.DECREASING, false);
Assert.fail("an exception should have been thrown");
} catch (NonMonotonicSequenceException e) {
// Expected
}
}
@Test
public void testIsMonotonic() {
Assert.assertFalse(MathArrays.isMonotonic(new double[] { -15, -5.5, -1, -1, 2, 15 },
MathArrays.OrderDirection.INCREASING, true));
Assert.assertTrue(MathArrays.isMonotonic(new double[] { -15, -5.5, -1, 0, 2, 15 },
MathArrays.OrderDirection.INCREASING, true));
Assert.assertFalse(MathArrays.isMonotonic(new double[] { -15, -5.5, -1, -2, 2 },
MathArrays.OrderDirection.INCREASING, false));
Assert.assertTrue(MathArrays.isMonotonic(new double[] { -15, -5.5, -1, -1, 2 },
MathArrays.OrderDirection.INCREASING, false));
Assert.assertFalse(MathArrays.isMonotonic(new double[] { 3, 3, -5.5, -11, -27.5 },
MathArrays.OrderDirection.DECREASING, true));
Assert.assertTrue(MathArrays.isMonotonic(new double[] { 3, 2, -5.5, -11, -27.5 },
MathArrays.OrderDirection.DECREASING, true));
Assert.assertFalse(MathArrays.isMonotonic(new double[] { 3, -1, 0, -5.5, -11, -27.5 },
MathArrays.OrderDirection.DECREASING, false));
Assert.assertTrue(MathArrays.isMonotonic(new double[] { 3, 0, 0, -5.5, -11, -27.5 },
MathArrays.OrderDirection.DECREASING, false));
}
@Test
public void testIsMonotonicComparable() {
Assert.assertFalse(MathArrays.isMonotonic(new Double[] { new Double(-15),
new Double(-5.5),
new Double(-1),
new Double(-1),
new Double(2),
new Double(15) },
MathArrays.OrderDirection.INCREASING, true));
Assert.assertTrue(MathArrays.isMonotonic(new Double[] { new Double(-15),
new Double(-5.5),
new Double(-1),
new Double(0),
new Double(2),
new Double(15) },
MathArrays.OrderDirection.INCREASING, true));
Assert.assertFalse(MathArrays.isMonotonic(new Double[] { new Double(-15),
new Double(-5.5),
new Double(-1),
new Double(-2),
new Double(2) },
MathArrays.OrderDirection.INCREASING, false));
Assert.assertTrue(MathArrays.isMonotonic(new Double[] { new Double(-15),
new Double(-5.5),
new Double(-1),
new Double(-1),
new Double(2) },
MathArrays.OrderDirection.INCREASING, false));
Assert.assertFalse(MathArrays.isMonotonic(new Double[] { new Double(3),
new Double(3),
new Double(-5.5),
new Double(-11),
new Double(-27.5) },
MathArrays.OrderDirection.DECREASING, true));
Assert.assertTrue(MathArrays.isMonotonic(new Double[] { new Double(3),
new Double(2),
new Double(-5.5),
new Double(-11),
new Double(-27.5) },
MathArrays.OrderDirection.DECREASING, true));
Assert.assertFalse(MathArrays.isMonotonic(new Double[] { new Double(3),
new Double(-1),
new Double(0),
new Double(-5.5),
new Double(-11),
new Double(-27.5) },
MathArrays.OrderDirection.DECREASING, false));
Assert.assertTrue(MathArrays.isMonotonic(new Double[] { new Double(3),
new Double(0),
new Double(0),
new Double(-5.5),
new Double(-11),
new Double(-27.5) },
MathArrays.OrderDirection.DECREASING, false));
}
@Test
public void testCheckRectangular() {
final long[][] rect = new long[][] {{0, 1}, {2, 3}};
final long[][] ragged = new long[][] {{0, 1}, {2}};
final long[][] nullArray = null;
final long[][] empty = new long[][] {};
MathArrays.checkRectangular(rect);
MathArrays.checkRectangular(empty);
try {
MathArrays.checkRectangular(ragged);
Assert.fail("Expecting DimensionMismatchException");
} catch (DimensionMismatchException ex) {
// Expected
}
try {
MathArrays.checkRectangular(nullArray);
Assert.fail("Expecting NullArgumentException");
} catch (NullArgumentException ex) {
// Expected
}
}
@Test
public void testCheckPositive() {
final double[] positive = new double[] {1, 2, 3};
final double[] nonNegative = new double[] {0, 1, 2};
final double[] nullArray = null;
final double[] empty = new double[] {};
MathArrays.checkPositive(positive);
MathArrays.checkPositive(empty);
try {
MathArrays.checkPositive(nullArray);
Assert.fail("Expecting NullPointerException");
} catch (NullPointerException ex) {
// Expected
}
try {
MathArrays.checkPositive(nonNegative);
Assert.fail("Expecting NotStrictlyPositiveException");
} catch (NotStrictlyPositiveException ex) {
// Expected
}
}
@Test
public void testCheckNonNegative() {
final long[] nonNegative = new long[] {0, 1};
final long[] hasNegative = new long[] {-1};
final long[] nullArray = null;
final long[] empty = new long[] {};
MathArrays.checkNonNegative(nonNegative);
MathArrays.checkNonNegative(empty);
try {
MathArrays.checkNonNegative(nullArray);
Assert.fail("Expecting NullPointerException");
} catch (NullPointerException ex) {
// Expected
}
try {
MathArrays.checkNonNegative(hasNegative);
Assert.fail("Expecting NotPositiveException");
} catch (NotPositiveException ex) {
// Expected
}
}
@Test
public void testCheckNonNegative2D() {
final long[][] nonNegative = new long[][] {{0, 1}, {1, 0}};
final long[][] hasNegative = new long[][] {{-1}, {0}};
final long[][] nullArray = null;
final long[][] empty = new long[][] {};
MathArrays.checkNonNegative(nonNegative);
MathArrays.checkNonNegative(empty);
try {
MathArrays.checkNonNegative(nullArray);
Assert.fail("Expecting NullPointerException");
} catch (NullPointerException ex) {
// Expected
}
try {
MathArrays.checkNonNegative(hasNegative);
Assert.fail("Expecting NotPositiveException");
} catch (NotPositiveException ex) {
// Expected
}
}
@Test
public void testSortInPlace() {
final double[] x1 = {2, 5, -3, 1, 4};
final double[] x2 = {4, 25, 9, 1, 16};
final double[] x3 = {8, 125, -27, 1, 64};
MathArrays.sortInPlace(x1, x2, x3);
Assert.assertEquals(-3, x1[0], Math.ulp(1d));
Assert.assertEquals(9, x2[0], Math.ulp(1d));
Assert.assertEquals(-27, x3[0], Math.ulp(1d));
Assert.assertEquals(1, x1[1], Math.ulp(1d));
Assert.assertEquals(1, x2[1], Math.ulp(1d));
Assert.assertEquals(1, x3[1], Math.ulp(1d));
Assert.assertEquals(2, x1[2], Math.ulp(1d));
Assert.assertEquals(4, x2[2], Math.ulp(1d));
Assert.assertEquals(8, x3[2], Math.ulp(1d));
Assert.assertEquals(4, x1[3], Math.ulp(1d));
Assert.assertEquals(16, x2[3], Math.ulp(1d));
Assert.assertEquals(64, x3[3], Math.ulp(1d));
Assert.assertEquals(5, x1[4], Math.ulp(1d));
Assert.assertEquals(25, x2[4], Math.ulp(1d));
Assert.assertEquals(125, x3[4], Math.ulp(1d));
}
@Test
public void testSortInPlaceDecresasingOrder() {
final double[] x1 = {2, 5, -3, 1, 4};
final double[] x2 = {4, 25, 9, 1, 16};
final double[] x3 = {8, 125, -27, 1, 64};
MathArrays.sortInPlace(x1,
MathArrays.OrderDirection.DECREASING,
x2, x3);
Assert.assertEquals(-3, x1[4], Math.ulp(1d));
Assert.assertEquals(9, x2[4], Math.ulp(1d));
Assert.assertEquals(-27, x3[4], Math.ulp(1d));
Assert.assertEquals(1, x1[3], Math.ulp(1d));
Assert.assertEquals(1, x2[3], Math.ulp(1d));
Assert.assertEquals(1, x3[3], Math.ulp(1d));
Assert.assertEquals(2, x1[2], Math.ulp(1d));
Assert.assertEquals(4, x2[2], Math.ulp(1d));
Assert.assertEquals(8, x3[2], Math.ulp(1d));
Assert.assertEquals(4, x1[1], Math.ulp(1d));
Assert.assertEquals(16, x2[1], Math.ulp(1d));
Assert.assertEquals(64, x3[1], Math.ulp(1d));
Assert.assertEquals(5, x1[0], Math.ulp(1d));
Assert.assertEquals(25, x2[0], Math.ulp(1d));
Assert.assertEquals(125, x3[0], Math.ulp(1d));
}
@Test
/** Example in javadoc */
public void testSortInPlaceExample() {
final double[] x = {3, 1, 2};
final double[] y = {1, 2, 3};
final double[] z = {0, 5, 7};
MathArrays.sortInPlace(x, y, z);
final double[] sx = {1, 2, 3};
final double[] sy = {2, 3, 1};
final double[] sz = {5, 7, 0};
Assert.assertTrue(Arrays.equals(sx, x));
Assert.assertTrue(Arrays.equals(sy, y));
Assert.assertTrue(Arrays.equals(sz, z));
}
@Test
public void testSortInPlaceFailures() {
final double[] nullArray = null;
final double[] one = {1};
final double[] two = {1, 2};
final double[] onep = {2};
try {
MathArrays.sortInPlace(one, two);
Assert.fail("Expecting DimensionMismatchException");
} catch (DimensionMismatchException ex) {
// expected
}
try {
MathArrays.sortInPlace(one, nullArray);
Assert.fail("Expecting NullArgumentException");
} catch (NullArgumentException ex) {
// expected
}
try {
MathArrays.sortInPlace(one, onep, nullArray);
Assert.fail("Expecting NullArgumentException");
} catch (NullArgumentException ex) {
// expected
}
}
@Test
public void testCopyOfInt() {
final int[] source = { Integer.MIN_VALUE,
-1, 0, 1, 3, 113, 4769,
Integer.MAX_VALUE };
final int[] dest = MathArrays.copyOf(source);
Assert.assertEquals(dest.length, source.length);
for (int i = 0; i < source.length; i++) {
Assert.assertEquals(source[i], dest[i]);
}
}
@Test
public void testCopyOfInt2() {
final int[] source = { Integer.MIN_VALUE,
-1, 0, 1, 3, 113, 4769,
Integer.MAX_VALUE };
final int offset = 3;
final int[] dest = MathArrays.copyOf(source, source.length - offset);
Assert.assertEquals(dest.length, source.length - offset);
for (int i = 0; i < source.length - offset; i++) {
Assert.assertEquals(source[i], dest[i]);
}
}
@Test
public void testCopyOfInt3() {
final int[] source = { Integer.MIN_VALUE,
-1, 0, 1, 3, 113, 4769,
Integer.MAX_VALUE };
final int offset = 3;
final int[] dest = MathArrays.copyOf(source, source.length + offset);
Assert.assertEquals(dest.length, source.length + offset);
for (int i = 0; i < source.length; i++) {
Assert.assertEquals(source[i], dest[i]);
}
for (int i = source.length; i < source.length + offset; i++) {
Assert.assertEquals(0, dest[i], 0);
}
}
@Test
public void testCopyOfDouble() {
final double[] source = { Double.NEGATIVE_INFINITY,
-Double.MAX_VALUE,
-1, 0,
Double.MIN_VALUE,
Math.ulp(1d),
1, 3, 113, 4769,
Double.MAX_VALUE,
Double.POSITIVE_INFINITY };
final double[] dest = MathArrays.copyOf(source);
Assert.assertEquals(dest.length, source.length);
for (int i = 0; i < source.length; i++) {
Assert.assertEquals(source[i], dest[i], 0);
}
}
@Test
public void testCopyOfDouble2() {
final double[] source = { Double.NEGATIVE_INFINITY,
-Double.MAX_VALUE,
-1, 0,
Double.MIN_VALUE,
Math.ulp(1d),
1, 3, 113, 4769,
Double.MAX_VALUE,
Double.POSITIVE_INFINITY };
final int offset = 3;
final double[] dest = MathArrays.copyOf(source, source.length - offset);
Assert.assertEquals(dest.length, source.length - offset);
for (int i = 0; i < source.length - offset; i++) {
Assert.assertEquals(source[i], dest[i], 0);
}
}
@Test
public void testCopyOfDouble3() {
final double[] source = { Double.NEGATIVE_INFINITY,
-Double.MAX_VALUE,
-1, 0,
Double.MIN_VALUE,
Math.ulp(1d),
1, 3, 113, 4769,
Double.MAX_VALUE,
Double.POSITIVE_INFINITY };
final int offset = 3;
final double[] dest = MathArrays.copyOf(source, source.length + offset);
Assert.assertEquals(dest.length, source.length + offset);
for (int i = 0; i < source.length; i++) {
Assert.assertEquals(source[i], dest[i], 0);
}
for (int i = source.length; i < source.length + offset; i++) {
Assert.assertEquals(0, dest[i], 0);
}
}
// MATH-1005
@Test
public void testLinearCombinationWithSingleElementArray() {
final double[] a = { 1.23456789 };
final double[] b = { 98765432.1 };
Assert.assertEquals(a[0] * b[0], MathArrays.linearCombination(a, b), 0d);
}
@Test
public void testLinearCombination1() {
final double[] a = new double[] {
-1321008684645961.0 / 268435456.0,
-5774608829631843.0 / 268435456.0,
-7645843051051357.0 / 8589934592.0
};
final double[] b = new double[] {
-5712344449280879.0 / 2097152.0,
-4550117129121957.0 / 2097152.0,
8846951984510141.0 / 131072.0
};
final double abSumInline = MathArrays.linearCombination(a[0], b[0],
a[1], b[1],
a[2], b[2]);
final double abSumArray = MathArrays.linearCombination(a, b);
Assert.assertEquals(abSumInline, abSumArray, 0);
Assert.assertEquals(-1.8551294182586248737720779899, abSumInline, 1.0e-15);
final double naive = a[0] * b[0] + a[1] * b[1] + a[2] * b[2];
Assert.assertTrue(FastMath.abs(naive - abSumInline) > 1.5);
}
@Test
public void testLinearCombination2() {
// we compare accurate versus naive dot product implementations
// on regular vectors (i.e. not extreme cases like in the previous test)
Well1024a random = new Well1024a(553267312521321234l);
for (int i = 0; i < 10000; ++i) {
final double ux = 1e17 * random.nextDouble();
final double uy = 1e17 * random.nextDouble();
final double uz = 1e17 * random.nextDouble();
final double vx = 1e17 * random.nextDouble();
final double vy = 1e17 * random.nextDouble();
final double vz = 1e17 * random.nextDouble();
final double sInline = MathArrays.linearCombination(ux, vx,
uy, vy,
uz, vz);
final double sArray = MathArrays.linearCombination(new double[] {ux, uy, uz},
new double[] {vx, vy, vz});
Assert.assertEquals(sInline, sArray, 0);
}
}
@Test
public void testLinearCombinationInfinite() {
final double[][] a = new double[][] {
{ 1, 2, 3, 4},
{ 1, Double.POSITIVE_INFINITY, 3, 4},
{ 1, 2, Double.POSITIVE_INFINITY, 4},
{ 1, Double.POSITIVE_INFINITY, 3, Double.NEGATIVE_INFINITY},
{ 1, 2, 3, 4},
{ 1, 2, 3, 4},
{ 1, 2, 3, 4},
{ 1, 2, 3, 4}
};
final double[][] b = new double[][] {
{ 1, -2, 3, 4},
{ 1, -2, 3, 4},
{ 1, -2, 3, 4},
{ 1, -2, 3, 4},
{ 1, Double.POSITIVE_INFINITY, 3, 4},
{ 1, -2, Double.POSITIVE_INFINITY, 4},
{ 1, Double.POSITIVE_INFINITY, 3, Double.NEGATIVE_INFINITY},
{ Double.NaN, -2, 3, 4}
};
Assert.assertEquals(-3,
MathArrays.linearCombination(a[0][0], b[0][0],
a[0][1], b[0][1]),
1.0e-10);
Assert.assertEquals(6,
MathArrays.linearCombination(a[0][0], b[0][0],
a[0][1], b[0][1],
a[0][2], b[0][2]),
1.0e-10);
Assert.assertEquals(22,
MathArrays.linearCombination(a[0][0], b[0][0],
a[0][1], b[0][1],
a[0][2], b[0][2],
a[0][3], b[0][3]),
1.0e-10);
Assert.assertEquals(22, MathArrays.linearCombination(a[0], b[0]), 1.0e-10);
Assert.assertEquals(Double.NEGATIVE_INFINITY,
MathArrays.linearCombination(a[1][0], b[1][0],
a[1][1], b[1][1]),
1.0e-10);
Assert.assertEquals(Double.NEGATIVE_INFINITY,
MathArrays.linearCombination(a[1][0], b[1][0],
a[1][1], b[1][1],
a[1][2], b[1][2]),
1.0e-10);
Assert.assertEquals(Double.NEGATIVE_INFINITY,
MathArrays.linearCombination(a[1][0], b[1][0],
a[1][1], b[1][1],
a[1][2], b[1][2],
a[1][3], b[1][3]),
1.0e-10);
Assert.assertEquals(Double.NEGATIVE_INFINITY, MathArrays.linearCombination(a[1], b[1]), 1.0e-10);
Assert.assertEquals(-3,
MathArrays.linearCombination(a[2][0], b[2][0],
a[2][1], b[2][1]),
1.0e-10);
Assert.assertEquals(Double.POSITIVE_INFINITY,
MathArrays.linearCombination(a[2][0], b[2][0],
a[2][1], b[2][1],
a[2][2], b[2][2]),
1.0e-10);
Assert.assertEquals(Double.POSITIVE_INFINITY,
MathArrays.linearCombination(a[2][0], b[2][0],
a[2][1], b[2][1],
a[2][2], b[2][2],
a[2][3], b[2][3]),
1.0e-10);
Assert.assertEquals(Double.POSITIVE_INFINITY, MathArrays.linearCombination(a[2], b[2]), 1.0e-10);
Assert.assertEquals(Double.NEGATIVE_INFINITY,
MathArrays.linearCombination(a[3][0], b[3][0],
a[3][1], b[3][1]),
1.0e-10);
Assert.assertEquals(Double.NEGATIVE_INFINITY,
MathArrays.linearCombination(a[3][0], b[3][0],
a[3][1], b[3][1],
a[3][2], b[3][2]),
1.0e-10);
Assert.assertEquals(Double.NEGATIVE_INFINITY,
MathArrays.linearCombination(a[3][0], b[3][0],
a[3][1], b[3][1],
a[3][2], b[3][2],
a[3][3], b[3][3]),
1.0e-10);
Assert.assertEquals(Double.NEGATIVE_INFINITY, MathArrays.linearCombination(a[3], b[3]), 1.0e-10);
Assert.assertEquals(Double.POSITIVE_INFINITY,
MathArrays.linearCombination(a[4][0], b[4][0],
a[4][1], b[4][1]),
1.0e-10);
Assert.assertEquals(Double.POSITIVE_INFINITY,
MathArrays.linearCombination(a[4][0], b[4][0],
a[4][1], b[4][1],
a[4][2], b[4][2]),
1.0e-10);
Assert.assertEquals(Double.POSITIVE_INFINITY,
MathArrays.linearCombination(a[4][0], b[4][0],
a[4][1], b[4][1],
a[4][2], b[4][2],
a[4][3], b[4][3]),
1.0e-10);
Assert.assertEquals(Double.POSITIVE_INFINITY, MathArrays.linearCombination(a[4], b[4]), 1.0e-10);
Assert.assertEquals(-3,
MathArrays.linearCombination(a[5][0], b[5][0],
a[5][1], b[5][1]),
1.0e-10);
Assert.assertEquals(Double.POSITIVE_INFINITY,
MathArrays.linearCombination(a[5][0], b[5][0],
a[5][1], b[5][1],
a[5][2], b[5][2]),
1.0e-10);
Assert.assertEquals(Double.POSITIVE_INFINITY,
MathArrays.linearCombination(a[5][0], b[5][0],
a[5][1], b[5][1],
a[5][2], b[5][2],
a[5][3], b[5][3]),
1.0e-10);
Assert.assertEquals(Double.POSITIVE_INFINITY, MathArrays.linearCombination(a[5], b[5]), 1.0e-10);
Assert.assertEquals(Double.POSITIVE_INFINITY,
MathArrays.linearCombination(a[6][0], b[6][0],
a[6][1], b[6][1]),
1.0e-10);
Assert.assertEquals(Double.POSITIVE_INFINITY,
MathArrays.linearCombination(a[6][0], b[6][0],
a[6][1], b[6][1],
a[6][2], b[6][2]),
1.0e-10);
Assert.assertTrue(Double.isNaN(MathArrays.linearCombination(a[6][0], b[6][0],
a[6][1], b[6][1],
a[6][2], b[6][2],
a[6][3], b[6][3])));
Assert.assertTrue(Double.isNaN(MathArrays.linearCombination(a[6], b[6])));
Assert.assertTrue(Double.isNaN(MathArrays.linearCombination(a[7][0], b[7][0],
a[7][1], b[7][1])));
Assert.assertTrue(Double.isNaN(MathArrays.linearCombination(a[7][0], b[7][0],
a[7][1], b[7][1],
a[7][2], b[7][2])));
Assert.assertTrue(Double.isNaN(MathArrays.linearCombination(a[7][0], b[7][0],
a[7][1], b[7][1],
a[7][2], b[7][2],
a[7][3], b[7][3])));
Assert.assertTrue(Double.isNaN(MathArrays.linearCombination(a[7], b[7])));
}
@Test
public void testArrayEquals() {
Assert.assertFalse(MathArrays.equals(new double[] { 1d }, null));
Assert.assertFalse(MathArrays.equals(null, new double[] { 1d }));
Assert.assertTrue(MathArrays.equals((double[]) null, (double[]) null));
Assert.assertFalse(MathArrays.equals(new double[] { 1d }, new double[0]));
Assert.assertTrue(MathArrays.equals(new double[] { 1d }, new double[] { 1d }));
Assert.assertTrue(MathArrays.equals(new double[] { Double.POSITIVE_INFINITY,
Double.NEGATIVE_INFINITY, 1d, 0d },
new double[] { Double.POSITIVE_INFINITY,
Double.NEGATIVE_INFINITY, 1d, 0d }));
Assert.assertFalse(MathArrays.equals(new double[] { Double.NaN },
new double[] { Double.NaN }));
Assert.assertFalse(MathArrays.equals(new double[] { Double.POSITIVE_INFINITY },
new double[] { Double.NEGATIVE_INFINITY }));
Assert.assertFalse(MathArrays.equals(new double[] { 1d },
new double[] { FastMath.nextAfter(FastMath.nextAfter(1d, 2d), 2d) }));
}
@Test
public void testArrayEqualsIncludingNaN() {
Assert.assertFalse(MathArrays.equalsIncludingNaN(new double[] { 1d }, null));
Assert.assertFalse(MathArrays.equalsIncludingNaN(null, new double[] { 1d }));
Assert.assertTrue(MathArrays.equalsIncludingNaN((double[]) null, (double[]) null));
Assert.assertFalse(MathArrays.equalsIncludingNaN(new double[] { 1d }, new double[0]));
Assert.assertTrue(MathArrays.equalsIncludingNaN(new double[] { 1d }, new double[] { 1d }));
Assert.assertTrue(MathArrays.equalsIncludingNaN(new double[] { Double.NaN, Double.POSITIVE_INFINITY,
Double.NEGATIVE_INFINITY, 1d, 0d },
new double[] { Double.NaN, Double.POSITIVE_INFINITY,
Double.NEGATIVE_INFINITY, 1d, 0d }));
Assert.assertFalse(MathArrays.equalsIncludingNaN(new double[] { Double.POSITIVE_INFINITY },
new double[] { Double.NEGATIVE_INFINITY }));
Assert.assertFalse(MathArrays.equalsIncludingNaN(new double[] { 1d },
new double[] { FastMath.nextAfter(FastMath.nextAfter(1d, 2d), 2d) }));
}
@Test
public void testNormalizeArray() {
double[] testValues1 = new double[] {1, 1, 2};
TestUtils.assertEquals( new double[] {.25, .25, .5},
MathArrays.normalizeArray(testValues1, 1),
Double.MIN_VALUE);
double[] testValues2 = new double[] {-1, -1, 1};
TestUtils.assertEquals( new double[] {1, 1, -1},
MathArrays.normalizeArray(testValues2, 1),
Double.MIN_VALUE);
// Ignore NaNs
double[] testValues3 = new double[] {-1, -1, Double.NaN, 1, Double.NaN};
TestUtils.assertEquals( new double[] {1, 1,Double.NaN, -1, Double.NaN},
MathArrays.normalizeArray(testValues3, 1),
Double.MIN_VALUE);
// Zero sum -> MathArithmeticException
double[] zeroSum = new double[] {-1, 1};
try {
MathArrays.normalizeArray(zeroSum, 1);
Assert.fail("expecting MathArithmeticException");
} catch (MathArithmeticException ex) {}
// Infinite elements -> MathArithmeticException
double[] hasInf = new double[] {1, 2, 1, Double.NEGATIVE_INFINITY};
try {
MathArrays.normalizeArray(hasInf, 1);
Assert.fail("expecting MathIllegalArgumentException");
} catch (MathIllegalArgumentException ex) {}
// Infinite target -> MathIllegalArgumentException
try {
MathArrays.normalizeArray(testValues1, Double.POSITIVE_INFINITY);
Assert.fail("expecting MathIllegalArgumentException");
} catch (MathIllegalArgumentException ex) {}
// NaN target -> MathIllegalArgumentException
try {
MathArrays.normalizeArray(testValues1, Double.NaN);
Assert.fail("expecting MathIllegalArgumentException");
} catch (MathIllegalArgumentException ex) {}
}
@Test
public void testConvolve() {
/* Test Case (obtained via SciPy)
* x=[1.2,-1.8,1.4]
* h=[1,0.8,0.5,0.3]
* convolve(x,h) -> array([ 1.2 , -0.84, 0.56, 0.58, 0.16, 0.42])
*/
double[] x1 = { 1.2, -1.8, 1.4 };
double[] h1 = { 1, 0.8, 0.5, 0.3 };
double[] y1 = { 1.2, -0.84, 0.56, 0.58, 0.16, 0.42 };
double tolerance = 1e-13;
double[] yActual = MathArrays.convolve(x1, h1);
Assert.assertArrayEquals(y1, yActual, tolerance);
double[] x2 = { 1, 2, 3 };
double[] h2 = { 0, 1, 0.5 };
double[] y2 = { 0, 1, 2.5, 4, 1.5 };
yActual = MathArrays.convolve(x2, h2);
Assert.assertArrayEquals(y2, yActual, tolerance);
try {
MathArrays.convolve(new double[]{1, 2}, null);
Assert.fail("an exception should have been thrown");
} catch (NullArgumentException e) {
// expected behavior
}
try {
MathArrays.convolve(null, new double[]{1, 2});
Assert.fail("an exception should have been thrown");
} catch (NullArgumentException e) {
// expected behavior
}
try {
MathArrays.convolve(new double[]{1, 2}, new double[]{});
Assert.fail("an exception should have been thrown");
} catch (NoDataException e) {
// expected behavior
}
try {
MathArrays.convolve(new double[]{}, new double[]{1, 2});
Assert.fail("an exception should have been thrown");
} catch (NoDataException e) {
// expected behavior
}
try {
MathArrays.convolve(new double[]{}, new double[]{});
Assert.fail("an exception should have been thrown");
} catch (NoDataException e) {
// expected behavior
}
}
} | // You are a professional Java test case writer, please create a test case named `testLinearCombinationWithSingleElementArray` for the issue `Math-MATH-1005`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Math-MATH-1005
//
// ## Issue-Title:
// ArrayIndexOutOfBoundsException in MathArrays.linearCombination
//
// ## Issue-Description:
//
// When MathArrays.linearCombination is passed arguments with length 1, it throws an ArrayOutOfBoundsException. This is caused by this line:
//
//
// double prodHighNext = prodHigh[1];
//
//
// linearCombination should check the length of the arguments and fall back to simple multiplication if length == 1.
//
//
//
//
//
@Test
public void testLinearCombinationWithSingleElementArray() {
| 591 | // MATH-1005 | 3 | 585 | src/test/java/org/apache/commons/math3/util/MathArraysTest.java | src/test/java | ```markdown
## Issue-ID: Math-MATH-1005
## Issue-Title:
ArrayIndexOutOfBoundsException in MathArrays.linearCombination
## Issue-Description:
When MathArrays.linearCombination is passed arguments with length 1, it throws an ArrayOutOfBoundsException. This is caused by this line:
double prodHighNext = prodHigh[1];
linearCombination should check the length of the arguments and fall back to simple multiplication if length == 1.
```
You are a professional Java test case writer, please create a test case named `testLinearCombinationWithSingleElementArray` for the issue `Math-MATH-1005`, utilizing the provided issue report information and the following function signature.
```java
@Test
public void testLinearCombinationWithSingleElementArray() {
```
| 585 | [
"org.apache.commons.math3.util.MathArrays"
] | 948ee0f50e84b33739a238cbc250ea91b578d5e791852aca1e27ff5fd5e4e008 | @Test
public void testLinearCombinationWithSingleElementArray() | // You are a professional Java test case writer, please create a test case named `testLinearCombinationWithSingleElementArray` for the issue `Math-MATH-1005`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Math-MATH-1005
//
// ## Issue-Title:
// ArrayIndexOutOfBoundsException in MathArrays.linearCombination
//
// ## Issue-Description:
//
// When MathArrays.linearCombination is passed arguments with length 1, it throws an ArrayOutOfBoundsException. This is caused by this line:
//
//
// double prodHighNext = prodHigh[1];
//
//
// linearCombination should check the length of the arguments and fall back to simple multiplication if length == 1.
//
//
//
//
//
| Math | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with this
* work for additional information regarding copyright ownership. The ASF
* licenses this file to You under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0 Unless required by applicable law
* or agreed to in writing, software distributed under the License is
* distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package org.apache.commons.math3.util;
import java.util.Arrays;
import org.apache.commons.math3.TestUtils;
import org.apache.commons.math3.exception.DimensionMismatchException;
import org.apache.commons.math3.exception.MathArithmeticException;
import org.apache.commons.math3.exception.MathIllegalArgumentException;
import org.apache.commons.math3.exception.NoDataException;
import org.apache.commons.math3.exception.NonMonotonicSequenceException;
import org.apache.commons.math3.exception.NotPositiveException;
import org.apache.commons.math3.exception.NotStrictlyPositiveException;
import org.apache.commons.math3.exception.NullArgumentException;
import org.apache.commons.math3.random.Well1024a;
import org.junit.Assert;
import org.junit.Test;
/**
* Test cases for the {@link MathArrays} class.
*
* @version $Id$
*/
public class MathArraysTest {
@Test
public void testScale() {
final double[] test = new double[] { -2.5, -1, 0, 1, 2.5 };
final double[] correctTest = MathArrays.copyOf(test);
final double[] correctScaled = new double[]{5.25, 2.1, 0, -2.1, -5.25};
final double[] scaled = MathArrays.scale(-2.1, test);
// Make sure test has not changed
for (int i = 0; i < test.length; i++) {
Assert.assertEquals(correctTest[i], test[i], 0);
}
// Test scaled values
for (int i = 0; i < scaled.length; i++) {
Assert.assertEquals(correctScaled[i], scaled[i], 0);
}
}
@Test
public void testScaleInPlace() {
final double[] test = new double[] { -2.5, -1, 0, 1, 2.5 };
final double[] correctScaled = new double[]{5.25, 2.1, 0, -2.1, -5.25};
MathArrays.scaleInPlace(-2.1, test);
// Make sure test has changed
for (int i = 0; i < test.length; i++) {
Assert.assertEquals(correctScaled[i], test[i], 0);
}
}
@Test(expected=DimensionMismatchException.class)
public void testEbeAddPrecondition() {
MathArrays.ebeAdd(new double[3], new double[4]);
}
@Test(expected=DimensionMismatchException.class)
public void testEbeSubtractPrecondition() {
MathArrays.ebeSubtract(new double[3], new double[4]);
}
@Test(expected=DimensionMismatchException.class)
public void testEbeMultiplyPrecondition() {
MathArrays.ebeMultiply(new double[3], new double[4]);
}
@Test(expected=DimensionMismatchException.class)
public void testEbeDividePrecondition() {
MathArrays.ebeDivide(new double[3], new double[4]);
}
@Test
public void testEbeAdd() {
final double[] a = { 0, 1, 2 };
final double[] b = { 3, 5, 7 };
final double[] r = MathArrays.ebeAdd(a, b);
for (int i = 0; i < a.length; i++) {
Assert.assertEquals(a[i] + b[i], r[i], 0);
}
}
@Test
public void testEbeSubtract() {
final double[] a = { 0, 1, 2 };
final double[] b = { 3, 5, 7 };
final double[] r = MathArrays.ebeSubtract(a, b);
for (int i = 0; i < a.length; i++) {
Assert.assertEquals(a[i] - b[i], r[i], 0);
}
}
@Test
public void testEbeMultiply() {
final double[] a = { 0, 1, 2 };
final double[] b = { 3, 5, 7 };
final double[] r = MathArrays.ebeMultiply(a, b);
for (int i = 0; i < a.length; i++) {
Assert.assertEquals(a[i] * b[i], r[i], 0);
}
}
@Test
public void testEbeDivide() {
final double[] a = { 0, 1, 2 };
final double[] b = { 3, 5, 7 };
final double[] r = MathArrays.ebeDivide(a, b);
for (int i = 0; i < a.length; i++) {
Assert.assertEquals(a[i] / b[i], r[i], 0);
}
}
@Test
public void testL1DistanceDouble() {
double[] p1 = { 2.5, 0.0 };
double[] p2 = { -0.5, 4.0 };
Assert.assertTrue(Precision.equals(7.0, MathArrays.distance1(p1, p2), 1));
}
@Test
public void testL1DistanceInt() {
int[] p1 = { 3, 0 };
int[] p2 = { 0, 4 };
Assert.assertEquals(7, MathArrays.distance1(p1, p2));
}
@Test
public void testL2DistanceDouble() {
double[] p1 = { 2.5, 0.0 };
double[] p2 = { -0.5, 4.0 };
Assert.assertTrue(Precision.equals(5.0, MathArrays.distance(p1, p2), 1));
}
@Test
public void testL2DistanceInt() {
int[] p1 = { 3, 0 };
int[] p2 = { 0, 4 };
Assert.assertTrue(Precision.equals(5, MathArrays.distance(p1, p2), 1));
}
@Test
public void testLInfDistanceDouble() {
double[] p1 = { 2.5, 0.0 };
double[] p2 = { -0.5, 4.0 };
Assert.assertTrue(Precision.equals(4.0, MathArrays.distanceInf(p1, p2), 1));
}
@Test
public void testLInfDistanceInt() {
int[] p1 = { 3, 0 };
int[] p2 = { 0, 4 };
Assert.assertEquals(4, MathArrays.distanceInf(p1, p2));
}
@Test
public void testCheckOrder() {
MathArrays.checkOrder(new double[] {-15, -5.5, -1, 2, 15},
MathArrays.OrderDirection.INCREASING, true);
MathArrays.checkOrder(new double[] {-15, -5.5, -1, 2, 2},
MathArrays.OrderDirection.INCREASING, false);
MathArrays.checkOrder(new double[] {3, -5.5, -11, -27.5},
MathArrays.OrderDirection.DECREASING, true);
MathArrays.checkOrder(new double[] {3, 0, 0, -5.5, -11, -27.5},
MathArrays.OrderDirection.DECREASING, false);
try {
MathArrays.checkOrder(new double[] {-15, -5.5, -1, -1, 2, 15},
MathArrays.OrderDirection.INCREASING, true);
Assert.fail("an exception should have been thrown");
} catch (NonMonotonicSequenceException e) {
// Expected
}
try {
MathArrays.checkOrder(new double[] {-15, -5.5, -1, -2, 2},
MathArrays.OrderDirection.INCREASING, false);
Assert.fail("an exception should have been thrown");
} catch (NonMonotonicSequenceException e) {
// Expected
}
try {
MathArrays.checkOrder(new double[] {3, 3, -5.5, -11, -27.5},
MathArrays.OrderDirection.DECREASING, true);
Assert.fail("an exception should have been thrown");
} catch (NonMonotonicSequenceException e) {
// Expected
}
try {
MathArrays.checkOrder(new double[] {3, -1, 0, -5.5, -11, -27.5},
MathArrays.OrderDirection.DECREASING, false);
Assert.fail("an exception should have been thrown");
} catch (NonMonotonicSequenceException e) {
// Expected
}
try {
MathArrays.checkOrder(new double[] {3, 0, -5.5, -11, -10},
MathArrays.OrderDirection.DECREASING, false);
Assert.fail("an exception should have been thrown");
} catch (NonMonotonicSequenceException e) {
// Expected
}
}
@Test
public void testIsMonotonic() {
Assert.assertFalse(MathArrays.isMonotonic(new double[] { -15, -5.5, -1, -1, 2, 15 },
MathArrays.OrderDirection.INCREASING, true));
Assert.assertTrue(MathArrays.isMonotonic(new double[] { -15, -5.5, -1, 0, 2, 15 },
MathArrays.OrderDirection.INCREASING, true));
Assert.assertFalse(MathArrays.isMonotonic(new double[] { -15, -5.5, -1, -2, 2 },
MathArrays.OrderDirection.INCREASING, false));
Assert.assertTrue(MathArrays.isMonotonic(new double[] { -15, -5.5, -1, -1, 2 },
MathArrays.OrderDirection.INCREASING, false));
Assert.assertFalse(MathArrays.isMonotonic(new double[] { 3, 3, -5.5, -11, -27.5 },
MathArrays.OrderDirection.DECREASING, true));
Assert.assertTrue(MathArrays.isMonotonic(new double[] { 3, 2, -5.5, -11, -27.5 },
MathArrays.OrderDirection.DECREASING, true));
Assert.assertFalse(MathArrays.isMonotonic(new double[] { 3, -1, 0, -5.5, -11, -27.5 },
MathArrays.OrderDirection.DECREASING, false));
Assert.assertTrue(MathArrays.isMonotonic(new double[] { 3, 0, 0, -5.5, -11, -27.5 },
MathArrays.OrderDirection.DECREASING, false));
}
@Test
public void testIsMonotonicComparable() {
Assert.assertFalse(MathArrays.isMonotonic(new Double[] { new Double(-15),
new Double(-5.5),
new Double(-1),
new Double(-1),
new Double(2),
new Double(15) },
MathArrays.OrderDirection.INCREASING, true));
Assert.assertTrue(MathArrays.isMonotonic(new Double[] { new Double(-15),
new Double(-5.5),
new Double(-1),
new Double(0),
new Double(2),
new Double(15) },
MathArrays.OrderDirection.INCREASING, true));
Assert.assertFalse(MathArrays.isMonotonic(new Double[] { new Double(-15),
new Double(-5.5),
new Double(-1),
new Double(-2),
new Double(2) },
MathArrays.OrderDirection.INCREASING, false));
Assert.assertTrue(MathArrays.isMonotonic(new Double[] { new Double(-15),
new Double(-5.5),
new Double(-1),
new Double(-1),
new Double(2) },
MathArrays.OrderDirection.INCREASING, false));
Assert.assertFalse(MathArrays.isMonotonic(new Double[] { new Double(3),
new Double(3),
new Double(-5.5),
new Double(-11),
new Double(-27.5) },
MathArrays.OrderDirection.DECREASING, true));
Assert.assertTrue(MathArrays.isMonotonic(new Double[] { new Double(3),
new Double(2),
new Double(-5.5),
new Double(-11),
new Double(-27.5) },
MathArrays.OrderDirection.DECREASING, true));
Assert.assertFalse(MathArrays.isMonotonic(new Double[] { new Double(3),
new Double(-1),
new Double(0),
new Double(-5.5),
new Double(-11),
new Double(-27.5) },
MathArrays.OrderDirection.DECREASING, false));
Assert.assertTrue(MathArrays.isMonotonic(new Double[] { new Double(3),
new Double(0),
new Double(0),
new Double(-5.5),
new Double(-11),
new Double(-27.5) },
MathArrays.OrderDirection.DECREASING, false));
}
@Test
public void testCheckRectangular() {
final long[][] rect = new long[][] {{0, 1}, {2, 3}};
final long[][] ragged = new long[][] {{0, 1}, {2}};
final long[][] nullArray = null;
final long[][] empty = new long[][] {};
MathArrays.checkRectangular(rect);
MathArrays.checkRectangular(empty);
try {
MathArrays.checkRectangular(ragged);
Assert.fail("Expecting DimensionMismatchException");
} catch (DimensionMismatchException ex) {
// Expected
}
try {
MathArrays.checkRectangular(nullArray);
Assert.fail("Expecting NullArgumentException");
} catch (NullArgumentException ex) {
// Expected
}
}
@Test
public void testCheckPositive() {
final double[] positive = new double[] {1, 2, 3};
final double[] nonNegative = new double[] {0, 1, 2};
final double[] nullArray = null;
final double[] empty = new double[] {};
MathArrays.checkPositive(positive);
MathArrays.checkPositive(empty);
try {
MathArrays.checkPositive(nullArray);
Assert.fail("Expecting NullPointerException");
} catch (NullPointerException ex) {
// Expected
}
try {
MathArrays.checkPositive(nonNegative);
Assert.fail("Expecting NotStrictlyPositiveException");
} catch (NotStrictlyPositiveException ex) {
// Expected
}
}
@Test
public void testCheckNonNegative() {
final long[] nonNegative = new long[] {0, 1};
final long[] hasNegative = new long[] {-1};
final long[] nullArray = null;
final long[] empty = new long[] {};
MathArrays.checkNonNegative(nonNegative);
MathArrays.checkNonNegative(empty);
try {
MathArrays.checkNonNegative(nullArray);
Assert.fail("Expecting NullPointerException");
} catch (NullPointerException ex) {
// Expected
}
try {
MathArrays.checkNonNegative(hasNegative);
Assert.fail("Expecting NotPositiveException");
} catch (NotPositiveException ex) {
// Expected
}
}
@Test
public void testCheckNonNegative2D() {
final long[][] nonNegative = new long[][] {{0, 1}, {1, 0}};
final long[][] hasNegative = new long[][] {{-1}, {0}};
final long[][] nullArray = null;
final long[][] empty = new long[][] {};
MathArrays.checkNonNegative(nonNegative);
MathArrays.checkNonNegative(empty);
try {
MathArrays.checkNonNegative(nullArray);
Assert.fail("Expecting NullPointerException");
} catch (NullPointerException ex) {
// Expected
}
try {
MathArrays.checkNonNegative(hasNegative);
Assert.fail("Expecting NotPositiveException");
} catch (NotPositiveException ex) {
// Expected
}
}
@Test
public void testSortInPlace() {
final double[] x1 = {2, 5, -3, 1, 4};
final double[] x2 = {4, 25, 9, 1, 16};
final double[] x3 = {8, 125, -27, 1, 64};
MathArrays.sortInPlace(x1, x2, x3);
Assert.assertEquals(-3, x1[0], Math.ulp(1d));
Assert.assertEquals(9, x2[0], Math.ulp(1d));
Assert.assertEquals(-27, x3[0], Math.ulp(1d));
Assert.assertEquals(1, x1[1], Math.ulp(1d));
Assert.assertEquals(1, x2[1], Math.ulp(1d));
Assert.assertEquals(1, x3[1], Math.ulp(1d));
Assert.assertEquals(2, x1[2], Math.ulp(1d));
Assert.assertEquals(4, x2[2], Math.ulp(1d));
Assert.assertEquals(8, x3[2], Math.ulp(1d));
Assert.assertEquals(4, x1[3], Math.ulp(1d));
Assert.assertEquals(16, x2[3], Math.ulp(1d));
Assert.assertEquals(64, x3[3], Math.ulp(1d));
Assert.assertEquals(5, x1[4], Math.ulp(1d));
Assert.assertEquals(25, x2[4], Math.ulp(1d));
Assert.assertEquals(125, x3[4], Math.ulp(1d));
}
@Test
public void testSortInPlaceDecresasingOrder() {
final double[] x1 = {2, 5, -3, 1, 4};
final double[] x2 = {4, 25, 9, 1, 16};
final double[] x3 = {8, 125, -27, 1, 64};
MathArrays.sortInPlace(x1,
MathArrays.OrderDirection.DECREASING,
x2, x3);
Assert.assertEquals(-3, x1[4], Math.ulp(1d));
Assert.assertEquals(9, x2[4], Math.ulp(1d));
Assert.assertEquals(-27, x3[4], Math.ulp(1d));
Assert.assertEquals(1, x1[3], Math.ulp(1d));
Assert.assertEquals(1, x2[3], Math.ulp(1d));
Assert.assertEquals(1, x3[3], Math.ulp(1d));
Assert.assertEquals(2, x1[2], Math.ulp(1d));
Assert.assertEquals(4, x2[2], Math.ulp(1d));
Assert.assertEquals(8, x3[2], Math.ulp(1d));
Assert.assertEquals(4, x1[1], Math.ulp(1d));
Assert.assertEquals(16, x2[1], Math.ulp(1d));
Assert.assertEquals(64, x3[1], Math.ulp(1d));
Assert.assertEquals(5, x1[0], Math.ulp(1d));
Assert.assertEquals(25, x2[0], Math.ulp(1d));
Assert.assertEquals(125, x3[0], Math.ulp(1d));
}
@Test
/** Example in javadoc */
public void testSortInPlaceExample() {
final double[] x = {3, 1, 2};
final double[] y = {1, 2, 3};
final double[] z = {0, 5, 7};
MathArrays.sortInPlace(x, y, z);
final double[] sx = {1, 2, 3};
final double[] sy = {2, 3, 1};
final double[] sz = {5, 7, 0};
Assert.assertTrue(Arrays.equals(sx, x));
Assert.assertTrue(Arrays.equals(sy, y));
Assert.assertTrue(Arrays.equals(sz, z));
}
@Test
public void testSortInPlaceFailures() {
final double[] nullArray = null;
final double[] one = {1};
final double[] two = {1, 2};
final double[] onep = {2};
try {
MathArrays.sortInPlace(one, two);
Assert.fail("Expecting DimensionMismatchException");
} catch (DimensionMismatchException ex) {
// expected
}
try {
MathArrays.sortInPlace(one, nullArray);
Assert.fail("Expecting NullArgumentException");
} catch (NullArgumentException ex) {
// expected
}
try {
MathArrays.sortInPlace(one, onep, nullArray);
Assert.fail("Expecting NullArgumentException");
} catch (NullArgumentException ex) {
// expected
}
}
@Test
public void testCopyOfInt() {
final int[] source = { Integer.MIN_VALUE,
-1, 0, 1, 3, 113, 4769,
Integer.MAX_VALUE };
final int[] dest = MathArrays.copyOf(source);
Assert.assertEquals(dest.length, source.length);
for (int i = 0; i < source.length; i++) {
Assert.assertEquals(source[i], dest[i]);
}
}
@Test
public void testCopyOfInt2() {
final int[] source = { Integer.MIN_VALUE,
-1, 0, 1, 3, 113, 4769,
Integer.MAX_VALUE };
final int offset = 3;
final int[] dest = MathArrays.copyOf(source, source.length - offset);
Assert.assertEquals(dest.length, source.length - offset);
for (int i = 0; i < source.length - offset; i++) {
Assert.assertEquals(source[i], dest[i]);
}
}
@Test
public void testCopyOfInt3() {
final int[] source = { Integer.MIN_VALUE,
-1, 0, 1, 3, 113, 4769,
Integer.MAX_VALUE };
final int offset = 3;
final int[] dest = MathArrays.copyOf(source, source.length + offset);
Assert.assertEquals(dest.length, source.length + offset);
for (int i = 0; i < source.length; i++) {
Assert.assertEquals(source[i], dest[i]);
}
for (int i = source.length; i < source.length + offset; i++) {
Assert.assertEquals(0, dest[i], 0);
}
}
@Test
public void testCopyOfDouble() {
final double[] source = { Double.NEGATIVE_INFINITY,
-Double.MAX_VALUE,
-1, 0,
Double.MIN_VALUE,
Math.ulp(1d),
1, 3, 113, 4769,
Double.MAX_VALUE,
Double.POSITIVE_INFINITY };
final double[] dest = MathArrays.copyOf(source);
Assert.assertEquals(dest.length, source.length);
for (int i = 0; i < source.length; i++) {
Assert.assertEquals(source[i], dest[i], 0);
}
}
@Test
public void testCopyOfDouble2() {
final double[] source = { Double.NEGATIVE_INFINITY,
-Double.MAX_VALUE,
-1, 0,
Double.MIN_VALUE,
Math.ulp(1d),
1, 3, 113, 4769,
Double.MAX_VALUE,
Double.POSITIVE_INFINITY };
final int offset = 3;
final double[] dest = MathArrays.copyOf(source, source.length - offset);
Assert.assertEquals(dest.length, source.length - offset);
for (int i = 0; i < source.length - offset; i++) {
Assert.assertEquals(source[i], dest[i], 0);
}
}
@Test
public void testCopyOfDouble3() {
final double[] source = { Double.NEGATIVE_INFINITY,
-Double.MAX_VALUE,
-1, 0,
Double.MIN_VALUE,
Math.ulp(1d),
1, 3, 113, 4769,
Double.MAX_VALUE,
Double.POSITIVE_INFINITY };
final int offset = 3;
final double[] dest = MathArrays.copyOf(source, source.length + offset);
Assert.assertEquals(dest.length, source.length + offset);
for (int i = 0; i < source.length; i++) {
Assert.assertEquals(source[i], dest[i], 0);
}
for (int i = source.length; i < source.length + offset; i++) {
Assert.assertEquals(0, dest[i], 0);
}
}
// MATH-1005
@Test
public void testLinearCombinationWithSingleElementArray() {
final double[] a = { 1.23456789 };
final double[] b = { 98765432.1 };
Assert.assertEquals(a[0] * b[0], MathArrays.linearCombination(a, b), 0d);
}
@Test
public void testLinearCombination1() {
final double[] a = new double[] {
-1321008684645961.0 / 268435456.0,
-5774608829631843.0 / 268435456.0,
-7645843051051357.0 / 8589934592.0
};
final double[] b = new double[] {
-5712344449280879.0 / 2097152.0,
-4550117129121957.0 / 2097152.0,
8846951984510141.0 / 131072.0
};
final double abSumInline = MathArrays.linearCombination(a[0], b[0],
a[1], b[1],
a[2], b[2]);
final double abSumArray = MathArrays.linearCombination(a, b);
Assert.assertEquals(abSumInline, abSumArray, 0);
Assert.assertEquals(-1.8551294182586248737720779899, abSumInline, 1.0e-15);
final double naive = a[0] * b[0] + a[1] * b[1] + a[2] * b[2];
Assert.assertTrue(FastMath.abs(naive - abSumInline) > 1.5);
}
@Test
public void testLinearCombination2() {
// we compare accurate versus naive dot product implementations
// on regular vectors (i.e. not extreme cases like in the previous test)
Well1024a random = new Well1024a(553267312521321234l);
for (int i = 0; i < 10000; ++i) {
final double ux = 1e17 * random.nextDouble();
final double uy = 1e17 * random.nextDouble();
final double uz = 1e17 * random.nextDouble();
final double vx = 1e17 * random.nextDouble();
final double vy = 1e17 * random.nextDouble();
final double vz = 1e17 * random.nextDouble();
final double sInline = MathArrays.linearCombination(ux, vx,
uy, vy,
uz, vz);
final double sArray = MathArrays.linearCombination(new double[] {ux, uy, uz},
new double[] {vx, vy, vz});
Assert.assertEquals(sInline, sArray, 0);
}
}
@Test
public void testLinearCombinationInfinite() {
final double[][] a = new double[][] {
{ 1, 2, 3, 4},
{ 1, Double.POSITIVE_INFINITY, 3, 4},
{ 1, 2, Double.POSITIVE_INFINITY, 4},
{ 1, Double.POSITIVE_INFINITY, 3, Double.NEGATIVE_INFINITY},
{ 1, 2, 3, 4},
{ 1, 2, 3, 4},
{ 1, 2, 3, 4},
{ 1, 2, 3, 4}
};
final double[][] b = new double[][] {
{ 1, -2, 3, 4},
{ 1, -2, 3, 4},
{ 1, -2, 3, 4},
{ 1, -2, 3, 4},
{ 1, Double.POSITIVE_INFINITY, 3, 4},
{ 1, -2, Double.POSITIVE_INFINITY, 4},
{ 1, Double.POSITIVE_INFINITY, 3, Double.NEGATIVE_INFINITY},
{ Double.NaN, -2, 3, 4}
};
Assert.assertEquals(-3,
MathArrays.linearCombination(a[0][0], b[0][0],
a[0][1], b[0][1]),
1.0e-10);
Assert.assertEquals(6,
MathArrays.linearCombination(a[0][0], b[0][0],
a[0][1], b[0][1],
a[0][2], b[0][2]),
1.0e-10);
Assert.assertEquals(22,
MathArrays.linearCombination(a[0][0], b[0][0],
a[0][1], b[0][1],
a[0][2], b[0][2],
a[0][3], b[0][3]),
1.0e-10);
Assert.assertEquals(22, MathArrays.linearCombination(a[0], b[0]), 1.0e-10);
Assert.assertEquals(Double.NEGATIVE_INFINITY,
MathArrays.linearCombination(a[1][0], b[1][0],
a[1][1], b[1][1]),
1.0e-10);
Assert.assertEquals(Double.NEGATIVE_INFINITY,
MathArrays.linearCombination(a[1][0], b[1][0],
a[1][1], b[1][1],
a[1][2], b[1][2]),
1.0e-10);
Assert.assertEquals(Double.NEGATIVE_INFINITY,
MathArrays.linearCombination(a[1][0], b[1][0],
a[1][1], b[1][1],
a[1][2], b[1][2],
a[1][3], b[1][3]),
1.0e-10);
Assert.assertEquals(Double.NEGATIVE_INFINITY, MathArrays.linearCombination(a[1], b[1]), 1.0e-10);
Assert.assertEquals(-3,
MathArrays.linearCombination(a[2][0], b[2][0],
a[2][1], b[2][1]),
1.0e-10);
Assert.assertEquals(Double.POSITIVE_INFINITY,
MathArrays.linearCombination(a[2][0], b[2][0],
a[2][1], b[2][1],
a[2][2], b[2][2]),
1.0e-10);
Assert.assertEquals(Double.POSITIVE_INFINITY,
MathArrays.linearCombination(a[2][0], b[2][0],
a[2][1], b[2][1],
a[2][2], b[2][2],
a[2][3], b[2][3]),
1.0e-10);
Assert.assertEquals(Double.POSITIVE_INFINITY, MathArrays.linearCombination(a[2], b[2]), 1.0e-10);
Assert.assertEquals(Double.NEGATIVE_INFINITY,
MathArrays.linearCombination(a[3][0], b[3][0],
a[3][1], b[3][1]),
1.0e-10);
Assert.assertEquals(Double.NEGATIVE_INFINITY,
MathArrays.linearCombination(a[3][0], b[3][0],
a[3][1], b[3][1],
a[3][2], b[3][2]),
1.0e-10);
Assert.assertEquals(Double.NEGATIVE_INFINITY,
MathArrays.linearCombination(a[3][0], b[3][0],
a[3][1], b[3][1],
a[3][2], b[3][2],
a[3][3], b[3][3]),
1.0e-10);
Assert.assertEquals(Double.NEGATIVE_INFINITY, MathArrays.linearCombination(a[3], b[3]), 1.0e-10);
Assert.assertEquals(Double.POSITIVE_INFINITY,
MathArrays.linearCombination(a[4][0], b[4][0],
a[4][1], b[4][1]),
1.0e-10);
Assert.assertEquals(Double.POSITIVE_INFINITY,
MathArrays.linearCombination(a[4][0], b[4][0],
a[4][1], b[4][1],
a[4][2], b[4][2]),
1.0e-10);
Assert.assertEquals(Double.POSITIVE_INFINITY,
MathArrays.linearCombination(a[4][0], b[4][0],
a[4][1], b[4][1],
a[4][2], b[4][2],
a[4][3], b[4][3]),
1.0e-10);
Assert.assertEquals(Double.POSITIVE_INFINITY, MathArrays.linearCombination(a[4], b[4]), 1.0e-10);
Assert.assertEquals(-3,
MathArrays.linearCombination(a[5][0], b[5][0],
a[5][1], b[5][1]),
1.0e-10);
Assert.assertEquals(Double.POSITIVE_INFINITY,
MathArrays.linearCombination(a[5][0], b[5][0],
a[5][1], b[5][1],
a[5][2], b[5][2]),
1.0e-10);
Assert.assertEquals(Double.POSITIVE_INFINITY,
MathArrays.linearCombination(a[5][0], b[5][0],
a[5][1], b[5][1],
a[5][2], b[5][2],
a[5][3], b[5][3]),
1.0e-10);
Assert.assertEquals(Double.POSITIVE_INFINITY, MathArrays.linearCombination(a[5], b[5]), 1.0e-10);
Assert.assertEquals(Double.POSITIVE_INFINITY,
MathArrays.linearCombination(a[6][0], b[6][0],
a[6][1], b[6][1]),
1.0e-10);
Assert.assertEquals(Double.POSITIVE_INFINITY,
MathArrays.linearCombination(a[6][0], b[6][0],
a[6][1], b[6][1],
a[6][2], b[6][2]),
1.0e-10);
Assert.assertTrue(Double.isNaN(MathArrays.linearCombination(a[6][0], b[6][0],
a[6][1], b[6][1],
a[6][2], b[6][2],
a[6][3], b[6][3])));
Assert.assertTrue(Double.isNaN(MathArrays.linearCombination(a[6], b[6])));
Assert.assertTrue(Double.isNaN(MathArrays.linearCombination(a[7][0], b[7][0],
a[7][1], b[7][1])));
Assert.assertTrue(Double.isNaN(MathArrays.linearCombination(a[7][0], b[7][0],
a[7][1], b[7][1],
a[7][2], b[7][2])));
Assert.assertTrue(Double.isNaN(MathArrays.linearCombination(a[7][0], b[7][0],
a[7][1], b[7][1],
a[7][2], b[7][2],
a[7][3], b[7][3])));
Assert.assertTrue(Double.isNaN(MathArrays.linearCombination(a[7], b[7])));
}
@Test
public void testArrayEquals() {
Assert.assertFalse(MathArrays.equals(new double[] { 1d }, null));
Assert.assertFalse(MathArrays.equals(null, new double[] { 1d }));
Assert.assertTrue(MathArrays.equals((double[]) null, (double[]) null));
Assert.assertFalse(MathArrays.equals(new double[] { 1d }, new double[0]));
Assert.assertTrue(MathArrays.equals(new double[] { 1d }, new double[] { 1d }));
Assert.assertTrue(MathArrays.equals(new double[] { Double.POSITIVE_INFINITY,
Double.NEGATIVE_INFINITY, 1d, 0d },
new double[] { Double.POSITIVE_INFINITY,
Double.NEGATIVE_INFINITY, 1d, 0d }));
Assert.assertFalse(MathArrays.equals(new double[] { Double.NaN },
new double[] { Double.NaN }));
Assert.assertFalse(MathArrays.equals(new double[] { Double.POSITIVE_INFINITY },
new double[] { Double.NEGATIVE_INFINITY }));
Assert.assertFalse(MathArrays.equals(new double[] { 1d },
new double[] { FastMath.nextAfter(FastMath.nextAfter(1d, 2d), 2d) }));
}
@Test
public void testArrayEqualsIncludingNaN() {
Assert.assertFalse(MathArrays.equalsIncludingNaN(new double[] { 1d }, null));
Assert.assertFalse(MathArrays.equalsIncludingNaN(null, new double[] { 1d }));
Assert.assertTrue(MathArrays.equalsIncludingNaN((double[]) null, (double[]) null));
Assert.assertFalse(MathArrays.equalsIncludingNaN(new double[] { 1d }, new double[0]));
Assert.assertTrue(MathArrays.equalsIncludingNaN(new double[] { 1d }, new double[] { 1d }));
Assert.assertTrue(MathArrays.equalsIncludingNaN(new double[] { Double.NaN, Double.POSITIVE_INFINITY,
Double.NEGATIVE_INFINITY, 1d, 0d },
new double[] { Double.NaN, Double.POSITIVE_INFINITY,
Double.NEGATIVE_INFINITY, 1d, 0d }));
Assert.assertFalse(MathArrays.equalsIncludingNaN(new double[] { Double.POSITIVE_INFINITY },
new double[] { Double.NEGATIVE_INFINITY }));
Assert.assertFalse(MathArrays.equalsIncludingNaN(new double[] { 1d },
new double[] { FastMath.nextAfter(FastMath.nextAfter(1d, 2d), 2d) }));
}
@Test
public void testNormalizeArray() {
double[] testValues1 = new double[] {1, 1, 2};
TestUtils.assertEquals( new double[] {.25, .25, .5},
MathArrays.normalizeArray(testValues1, 1),
Double.MIN_VALUE);
double[] testValues2 = new double[] {-1, -1, 1};
TestUtils.assertEquals( new double[] {1, 1, -1},
MathArrays.normalizeArray(testValues2, 1),
Double.MIN_VALUE);
// Ignore NaNs
double[] testValues3 = new double[] {-1, -1, Double.NaN, 1, Double.NaN};
TestUtils.assertEquals( new double[] {1, 1,Double.NaN, -1, Double.NaN},
MathArrays.normalizeArray(testValues3, 1),
Double.MIN_VALUE);
// Zero sum -> MathArithmeticException
double[] zeroSum = new double[] {-1, 1};
try {
MathArrays.normalizeArray(zeroSum, 1);
Assert.fail("expecting MathArithmeticException");
} catch (MathArithmeticException ex) {}
// Infinite elements -> MathArithmeticException
double[] hasInf = new double[] {1, 2, 1, Double.NEGATIVE_INFINITY};
try {
MathArrays.normalizeArray(hasInf, 1);
Assert.fail("expecting MathIllegalArgumentException");
} catch (MathIllegalArgumentException ex) {}
// Infinite target -> MathIllegalArgumentException
try {
MathArrays.normalizeArray(testValues1, Double.POSITIVE_INFINITY);
Assert.fail("expecting MathIllegalArgumentException");
} catch (MathIllegalArgumentException ex) {}
// NaN target -> MathIllegalArgumentException
try {
MathArrays.normalizeArray(testValues1, Double.NaN);
Assert.fail("expecting MathIllegalArgumentException");
} catch (MathIllegalArgumentException ex) {}
}
@Test
public void testConvolve() {
/* Test Case (obtained via SciPy)
* x=[1.2,-1.8,1.4]
* h=[1,0.8,0.5,0.3]
* convolve(x,h) -> array([ 1.2 , -0.84, 0.56, 0.58, 0.16, 0.42])
*/
double[] x1 = { 1.2, -1.8, 1.4 };
double[] h1 = { 1, 0.8, 0.5, 0.3 };
double[] y1 = { 1.2, -0.84, 0.56, 0.58, 0.16, 0.42 };
double tolerance = 1e-13;
double[] yActual = MathArrays.convolve(x1, h1);
Assert.assertArrayEquals(y1, yActual, tolerance);
double[] x2 = { 1, 2, 3 };
double[] h2 = { 0, 1, 0.5 };
double[] y2 = { 0, 1, 2.5, 4, 1.5 };
yActual = MathArrays.convolve(x2, h2);
Assert.assertArrayEquals(y2, yActual, tolerance);
try {
MathArrays.convolve(new double[]{1, 2}, null);
Assert.fail("an exception should have been thrown");
} catch (NullArgumentException e) {
// expected behavior
}
try {
MathArrays.convolve(null, new double[]{1, 2});
Assert.fail("an exception should have been thrown");
} catch (NullArgumentException e) {
// expected behavior
}
try {
MathArrays.convolve(new double[]{1, 2}, new double[]{});
Assert.fail("an exception should have been thrown");
} catch (NoDataException e) {
// expected behavior
}
try {
MathArrays.convolve(new double[]{}, new double[]{1, 2});
Assert.fail("an exception should have been thrown");
} catch (NoDataException e) {
// expected behavior
}
try {
MathArrays.convolve(new double[]{}, new double[]{});
Assert.fail("an exception should have been thrown");
} catch (NoDataException e) {
// expected behavior
}
}
} |
|
@Test(expected = ParseException.class)
public void testCreateValueInteger_failure()
throws Exception
{
TypeHandler.createValue("just-a-string", Integer.class);
} | org.apache.commons.cli.TypeHandlerTest::testCreateValueInteger_failure | src/test/java/org/apache/commons/cli/TypeHandlerTest.java | 155 | src/test/java/org/apache/commons/cli/TypeHandlerTest.java | testCreateValueInteger_failure | /**
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.cli;
import org.junit.Test;
import java.io.File;
import java.io.FileInputStream;
import java.net.URL;
import static org.junit.Assert.assertEquals;
import static org.junit.Assert.assertNotNull;
import static org.junit.Assert.assertTrue;
public class TypeHandlerTest
{
@Test
public void testCreateValueString()
throws Exception
{
assertEquals("String", TypeHandler.createValue("String", PatternOptionBuilder.STRING_VALUE));
}
@Test(expected = ParseException.class)
public void testCreateValueObject_unknownClass()
throws Exception
{
TypeHandler.createValue("unknown", PatternOptionBuilder.OBJECT_VALUE);
}
@Test(expected = ParseException.class)
public void testCreateValueObject_notInstantiableClass()
throws Exception
{
TypeHandler.createValue(NotInstantiable.class.getName(), PatternOptionBuilder.OBJECT_VALUE);
}
@Test
public void testCreateValueObject_InstantiableClass()
throws Exception
{
Object result = TypeHandler.createValue(Instantiable.class.getName(), PatternOptionBuilder.OBJECT_VALUE);
assertTrue(result instanceof Instantiable);
}
@Test(expected = ParseException.class)
public void testCreateValueNumber_noNumber()
throws Exception
{
TypeHandler.createValue("not a number", PatternOptionBuilder.NUMBER_VALUE);
}
@Test
public void testCreateValueNumber_Double()
throws Exception
{
assertEquals(1.5d, TypeHandler.createValue("1.5", PatternOptionBuilder.NUMBER_VALUE));
}
@Test
public void testCreateValueNumber_Long()
throws Exception
{
assertEquals(Long.valueOf(15), TypeHandler.createValue("15", PatternOptionBuilder.NUMBER_VALUE));
}
@Test(expected = UnsupportedOperationException.class)
public void testCreateValueDate()
throws Exception
{
TypeHandler.createValue("what ever", PatternOptionBuilder.DATE_VALUE);
}
@Test(expected = ParseException.class)
public void testCreateValueClass_notFound()
throws Exception
{
TypeHandler.createValue("what ever", PatternOptionBuilder.CLASS_VALUE);
}
@Test
public void testCreateValueClass()
throws Exception
{
Object clazz = TypeHandler.createValue(Instantiable.class.getName(), PatternOptionBuilder.CLASS_VALUE);
assertEquals(Instantiable.class, clazz);
}
@Test
public void testCreateValueFile()
throws Exception
{
File result = TypeHandler.createValue("some-file.txt", PatternOptionBuilder.FILE_VALUE);
assertEquals("some-file.txt", result.getName());
}
@Test
public void testCreateValueExistingFile()
throws Exception
{
FileInputStream result = TypeHandler.createValue("src/test/resources/existing-readable.file", PatternOptionBuilder.EXISTING_FILE_VALUE);
assertNotNull(result);
}
@Test(expected = ParseException.class)
public void testCreateValueExistingFile_nonExistingFile()
throws Exception
{
TypeHandler.createValue("non-existing.file", PatternOptionBuilder.EXISTING_FILE_VALUE);
}
@Test(expected = UnsupportedOperationException.class)
public void testCreateValueFiles()
throws Exception
{
TypeHandler.createValue("some.files", PatternOptionBuilder.FILES_VALUE);
}
@Test
public void testCreateValueURL()
throws Exception
{
String urlString = "http://commons.apache.org";
URL result = TypeHandler.createValue(urlString, PatternOptionBuilder.URL_VALUE);
assertEquals(urlString, result.toString());
}
@Test(expected = ParseException.class)
public void testCreateValueURL_malformed()
throws Exception
{
TypeHandler.createValue("malformed-url", PatternOptionBuilder.URL_VALUE);
}
@Test(expected = ParseException.class)
public void testCreateValueInteger_failure()
throws Exception
{
TypeHandler.createValue("just-a-string", Integer.class);
}
public static class Instantiable
{
}
public static class NotInstantiable
{
private NotInstantiable() {}
}
} | // You are a professional Java test case writer, please create a test case named `testCreateValueInteger_failure` for the issue `Cli-CLI-282`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Cli-CLI-282
//
// ## Issue-Title:
// TypeHandler should throw ParseException for an unsupported class
//
// ## Issue-Description:
//
// JavaDoc for TypeHandler states that createValue will
//
//
//
//
// ```
// * @throws ParseException if the value creation for the given object type failedtype
// ```
//
//
// However createValue(String str, Class<?> clazz) will return null if the clazz is unknown.
//
//
//
//
//
@Test(expected = ParseException.class)
public void testCreateValueInteger_failure()
throws Exception {
| 155 | 40 | 150 | src/test/java/org/apache/commons/cli/TypeHandlerTest.java | src/test/java | ```markdown
## Issue-ID: Cli-CLI-282
## Issue-Title:
TypeHandler should throw ParseException for an unsupported class
## Issue-Description:
JavaDoc for TypeHandler states that createValue will
```
* @throws ParseException if the value creation for the given object type failedtype
```
However createValue(String str, Class<?> clazz) will return null if the clazz is unknown.
```
You are a professional Java test case writer, please create a test case named `testCreateValueInteger_failure` for the issue `Cli-CLI-282`, utilizing the provided issue report information and the following function signature.
```java
@Test(expected = ParseException.class)
public void testCreateValueInteger_failure()
throws Exception {
```
| 150 | [
"org.apache.commons.cli.TypeHandler"
] | 9578e3d755df40483fc531f327018271915bf09e5af444ecacfd6f7520f0b972 | @Test(expected = ParseException.class)
public void testCreateValueInteger_failure()
throws Exception
| // You are a professional Java test case writer, please create a test case named `testCreateValueInteger_failure` for the issue `Cli-CLI-282`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Cli-CLI-282
//
// ## Issue-Title:
// TypeHandler should throw ParseException for an unsupported class
//
// ## Issue-Description:
//
// JavaDoc for TypeHandler states that createValue will
//
//
//
//
// ```
// * @throws ParseException if the value creation for the given object type failedtype
// ```
//
//
// However createValue(String str, Class<?> clazz) will return null if the clazz is unknown.
//
//
//
//
//
| Cli | /**
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.cli;
import org.junit.Test;
import java.io.File;
import java.io.FileInputStream;
import java.net.URL;
import static org.junit.Assert.assertEquals;
import static org.junit.Assert.assertNotNull;
import static org.junit.Assert.assertTrue;
public class TypeHandlerTest
{
@Test
public void testCreateValueString()
throws Exception
{
assertEquals("String", TypeHandler.createValue("String", PatternOptionBuilder.STRING_VALUE));
}
@Test(expected = ParseException.class)
public void testCreateValueObject_unknownClass()
throws Exception
{
TypeHandler.createValue("unknown", PatternOptionBuilder.OBJECT_VALUE);
}
@Test(expected = ParseException.class)
public void testCreateValueObject_notInstantiableClass()
throws Exception
{
TypeHandler.createValue(NotInstantiable.class.getName(), PatternOptionBuilder.OBJECT_VALUE);
}
@Test
public void testCreateValueObject_InstantiableClass()
throws Exception
{
Object result = TypeHandler.createValue(Instantiable.class.getName(), PatternOptionBuilder.OBJECT_VALUE);
assertTrue(result instanceof Instantiable);
}
@Test(expected = ParseException.class)
public void testCreateValueNumber_noNumber()
throws Exception
{
TypeHandler.createValue("not a number", PatternOptionBuilder.NUMBER_VALUE);
}
@Test
public void testCreateValueNumber_Double()
throws Exception
{
assertEquals(1.5d, TypeHandler.createValue("1.5", PatternOptionBuilder.NUMBER_VALUE));
}
@Test
public void testCreateValueNumber_Long()
throws Exception
{
assertEquals(Long.valueOf(15), TypeHandler.createValue("15", PatternOptionBuilder.NUMBER_VALUE));
}
@Test(expected = UnsupportedOperationException.class)
public void testCreateValueDate()
throws Exception
{
TypeHandler.createValue("what ever", PatternOptionBuilder.DATE_VALUE);
}
@Test(expected = ParseException.class)
public void testCreateValueClass_notFound()
throws Exception
{
TypeHandler.createValue("what ever", PatternOptionBuilder.CLASS_VALUE);
}
@Test
public void testCreateValueClass()
throws Exception
{
Object clazz = TypeHandler.createValue(Instantiable.class.getName(), PatternOptionBuilder.CLASS_VALUE);
assertEquals(Instantiable.class, clazz);
}
@Test
public void testCreateValueFile()
throws Exception
{
File result = TypeHandler.createValue("some-file.txt", PatternOptionBuilder.FILE_VALUE);
assertEquals("some-file.txt", result.getName());
}
@Test
public void testCreateValueExistingFile()
throws Exception
{
FileInputStream result = TypeHandler.createValue("src/test/resources/existing-readable.file", PatternOptionBuilder.EXISTING_FILE_VALUE);
assertNotNull(result);
}
@Test(expected = ParseException.class)
public void testCreateValueExistingFile_nonExistingFile()
throws Exception
{
TypeHandler.createValue("non-existing.file", PatternOptionBuilder.EXISTING_FILE_VALUE);
}
@Test(expected = UnsupportedOperationException.class)
public void testCreateValueFiles()
throws Exception
{
TypeHandler.createValue("some.files", PatternOptionBuilder.FILES_VALUE);
}
@Test
public void testCreateValueURL()
throws Exception
{
String urlString = "http://commons.apache.org";
URL result = TypeHandler.createValue(urlString, PatternOptionBuilder.URL_VALUE);
assertEquals(urlString, result.toString());
}
@Test(expected = ParseException.class)
public void testCreateValueURL_malformed()
throws Exception
{
TypeHandler.createValue("malformed-url", PatternOptionBuilder.URL_VALUE);
}
@Test(expected = ParseException.class)
public void testCreateValueInteger_failure()
throws Exception
{
TypeHandler.createValue("just-a-string", Integer.class);
}
public static class Instantiable
{
}
public static class NotInstantiable
{
private NotInstantiable() {}
}
} |
||
public void testXmlAttributesWithNextTextValue() throws Exception
{
final String XML = "<data max=\"7\" offset=\"9\"/>";
FromXmlParser xp = (FromXmlParser) _xmlFactory.createParser(new StringReader(XML));
// First: verify handling without forcing array handling:
assertToken(JsonToken.START_OBJECT, xp.nextToken()); // <data>
assertToken(JsonToken.FIELD_NAME, xp.nextToken()); // <max>
assertEquals("max", xp.getCurrentName());
assertEquals("7", xp.nextTextValue());
assertToken(JsonToken.FIELD_NAME, xp.nextToken()); // <offset>
assertEquals("offset", xp.getCurrentName());
assertEquals("offset", xp.getText());
assertEquals("9", xp.nextTextValue());
assertEquals("9", xp.getText());
assertToken(JsonToken.END_OBJECT, xp.nextToken()); // </data>
xp.close();
} | com.fasterxml.jackson.dataformat.xml.stream.XmlParserNextXxxTest::testXmlAttributesWithNextTextValue | src/test/java/com/fasterxml/jackson/dataformat/xml/stream/XmlParserNextXxxTest.java | 53 | src/test/java/com/fasterxml/jackson/dataformat/xml/stream/XmlParserNextXxxTest.java | testXmlAttributesWithNextTextValue | package com.fasterxml.jackson.dataformat.xml.stream;
import java.io.*;
import com.fasterxml.jackson.core.*;
import com.fasterxml.jackson.dataformat.xml.XmlFactory;
import com.fasterxml.jackson.dataformat.xml.XmlTestBase;
import com.fasterxml.jackson.dataformat.xml.deser.FromXmlParser;
public class XmlParserNextXxxTest extends XmlTestBase
{
protected JsonFactory _jsonFactory;
protected XmlFactory _xmlFactory;
// let's actually reuse XmlMapper to make things bit faster
@Override
public void setUp() throws Exception {
super.setUp();
_xmlFactory = new XmlFactory();
}
/*
/**********************************************************
/* Unit tests
/**********************************************************
*/
// [dataformat-xml#204]
public void testXmlAttributesWithNextTextValue() throws Exception
{
final String XML = "<data max=\"7\" offset=\"9\"/>";
FromXmlParser xp = (FromXmlParser) _xmlFactory.createParser(new StringReader(XML));
// First: verify handling without forcing array handling:
assertToken(JsonToken.START_OBJECT, xp.nextToken()); // <data>
assertToken(JsonToken.FIELD_NAME, xp.nextToken()); // <max>
assertEquals("max", xp.getCurrentName());
assertEquals("7", xp.nextTextValue());
assertToken(JsonToken.FIELD_NAME, xp.nextToken()); // <offset>
assertEquals("offset", xp.getCurrentName());
assertEquals("offset", xp.getText());
assertEquals("9", xp.nextTextValue());
assertEquals("9", xp.getText());
assertToken(JsonToken.END_OBJECT, xp.nextToken()); // </data>
xp.close();
}
} | // You are a professional Java test case writer, please create a test case named `testXmlAttributesWithNextTextValue` for the issue `JacksonXml-204`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonXml-204
//
// ## Issue-Title:
// FromXMLParser nextTextValue() incorrect for attributes
//
// ## Issue-Description:
// As of [#129](https://github.com/FasterXML/jackson-dataformat-xml/issues/129) the Method nextTextValue of FromXMLParser will no longer return a value for attributes. As the \_currToken is JsonToken.VALUE\_STRING in this case I think it is wrong to return null and it should return \_currText.
//
//
//
//
public void testXmlAttributesWithNextTextValue() throws Exception {
| 53 | // [dataformat-xml#204]
/*
/**********************************************************
/* Unit tests
/**********************************************************
*/ | 3 | 29 | src/test/java/com/fasterxml/jackson/dataformat/xml/stream/XmlParserNextXxxTest.java | src/test/java | ```markdown
## Issue-ID: JacksonXml-204
## Issue-Title:
FromXMLParser nextTextValue() incorrect for attributes
## Issue-Description:
As of [#129](https://github.com/FasterXML/jackson-dataformat-xml/issues/129) the Method nextTextValue of FromXMLParser will no longer return a value for attributes. As the \_currToken is JsonToken.VALUE\_STRING in this case I think it is wrong to return null and it should return \_currText.
```
You are a professional Java test case writer, please create a test case named `testXmlAttributesWithNextTextValue` for the issue `JacksonXml-204`, utilizing the provided issue report information and the following function signature.
```java
public void testXmlAttributesWithNextTextValue() throws Exception {
```
| 29 | [
"com.fasterxml.jackson.dataformat.xml.deser.FromXmlParser"
] | 958e58dc1fb7f5238b60d7f0efc923c50caaaddc8b148f36f6337d30dd610479 | public void testXmlAttributesWithNextTextValue() throws Exception
| // You are a professional Java test case writer, please create a test case named `testXmlAttributesWithNextTextValue` for the issue `JacksonXml-204`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonXml-204
//
// ## Issue-Title:
// FromXMLParser nextTextValue() incorrect for attributes
//
// ## Issue-Description:
// As of [#129](https://github.com/FasterXML/jackson-dataformat-xml/issues/129) the Method nextTextValue of FromXMLParser will no longer return a value for attributes. As the \_currToken is JsonToken.VALUE\_STRING in this case I think it is wrong to return null and it should return \_currText.
//
//
//
//
| JacksonXml | package com.fasterxml.jackson.dataformat.xml.stream;
import java.io.*;
import com.fasterxml.jackson.core.*;
import com.fasterxml.jackson.dataformat.xml.XmlFactory;
import com.fasterxml.jackson.dataformat.xml.XmlTestBase;
import com.fasterxml.jackson.dataformat.xml.deser.FromXmlParser;
public class XmlParserNextXxxTest extends XmlTestBase
{
protected JsonFactory _jsonFactory;
protected XmlFactory _xmlFactory;
// let's actually reuse XmlMapper to make things bit faster
@Override
public void setUp() throws Exception {
super.setUp();
_xmlFactory = new XmlFactory();
}
/*
/**********************************************************
/* Unit tests
/**********************************************************
*/
// [dataformat-xml#204]
public void testXmlAttributesWithNextTextValue() throws Exception
{
final String XML = "<data max=\"7\" offset=\"9\"/>";
FromXmlParser xp = (FromXmlParser) _xmlFactory.createParser(new StringReader(XML));
// First: verify handling without forcing array handling:
assertToken(JsonToken.START_OBJECT, xp.nextToken()); // <data>
assertToken(JsonToken.FIELD_NAME, xp.nextToken()); // <max>
assertEquals("max", xp.getCurrentName());
assertEquals("7", xp.nextTextValue());
assertToken(JsonToken.FIELD_NAME, xp.nextToken()); // <offset>
assertEquals("offset", xp.getCurrentName());
assertEquals("offset", xp.getText());
assertEquals("9", xp.nextTextValue());
assertEquals("9", xp.getText());
assertToken(JsonToken.END_OBJECT, xp.nextToken()); // </data>
xp.close();
}
} |
|
@Test
public void shouldAllowVerifyingWhenOtherMockCallIsInTheSameLine() {
//given
when(mock.otherMethod()).thenReturn("foo");
//when
mockTwo.simpleMethod("foo");
//then
verify(mockTwo).simpleMethod(mock.otherMethod());
try {
verify(mockTwo, never()).simpleMethod(mock.otherMethod());
fail();
} catch (NeverWantedButInvoked e) {}
} | org.mockitousage.bugs.VerifyingWithAnExtraCallToADifferentMockTest::shouldAllowVerifyingWhenOtherMockCallIsInTheSameLine | test/org/mockitousage/bugs/VerifyingWithAnExtraCallToADifferentMockTest.java | 34 | test/org/mockitousage/bugs/VerifyingWithAnExtraCallToADifferentMockTest.java | shouldAllowVerifyingWhenOtherMockCallIsInTheSameLine | /*
* Copyright (c) 2007 Mockito contributors
* This program is made available under the terms of the MIT License.
*/
package org.mockitousage.bugs;
import static org.mockito.Mockito.*;
import org.junit.Test;
import org.mockito.Mock;
import org.mockito.exceptions.verification.NeverWantedButInvoked;
import org.mockitousage.IMethods;
import org.mockitoutil.TestBase;
//see bug 138
public class VerifyingWithAnExtraCallToADifferentMockTest extends TestBase {
@Mock IMethods mock;
@Mock IMethods mockTwo;
@Test
public void shouldAllowVerifyingWhenOtherMockCallIsInTheSameLine() {
//given
when(mock.otherMethod()).thenReturn("foo");
//when
mockTwo.simpleMethod("foo");
//then
verify(mockTwo).simpleMethod(mock.otherMethod());
try {
verify(mockTwo, never()).simpleMethod(mock.otherMethod());
fail();
} catch (NeverWantedButInvoked e) {}
}
} | // You are a professional Java test case writer, please create a test case named `shouldAllowVerifyingWhenOtherMockCallIsInTheSameLine` for the issue `Mockito-138`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Mockito-138
//
// ## Issue-Title:
// fix proposal for #114
//
// ## Issue-Description:
// [@bric3](https://github.com/bric3), can you take a look at this one? If you don't have time I'll just merge it. All existing tests are passing.
//
//
// Thanks for the fix!!!
//
//
//
//
@Test
public void shouldAllowVerifyingWhenOtherMockCallIsInTheSameLine() {
| 34 | 13 | 20 | test/org/mockitousage/bugs/VerifyingWithAnExtraCallToADifferentMockTest.java | test | ```markdown
## Issue-ID: Mockito-138
## Issue-Title:
fix proposal for #114
## Issue-Description:
[@bric3](https://github.com/bric3), can you take a look at this one? If you don't have time I'll just merge it. All existing tests are passing.
Thanks for the fix!!!
```
You are a professional Java test case writer, please create a test case named `shouldAllowVerifyingWhenOtherMockCallIsInTheSameLine` for the issue `Mockito-138`, utilizing the provided issue report information and the following function signature.
```java
@Test
public void shouldAllowVerifyingWhenOtherMockCallIsInTheSameLine() {
```
| 20 | [
"org.mockito.internal.MockHandler"
] | 9785a86e564413e7642a35f96beee0e25e3c4ef7ccf957253f7c47ada7edb7de | @Test
public void shouldAllowVerifyingWhenOtherMockCallIsInTheSameLine() | // You are a professional Java test case writer, please create a test case named `shouldAllowVerifyingWhenOtherMockCallIsInTheSameLine` for the issue `Mockito-138`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Mockito-138
//
// ## Issue-Title:
// fix proposal for #114
//
// ## Issue-Description:
// [@bric3](https://github.com/bric3), can you take a look at this one? If you don't have time I'll just merge it. All existing tests are passing.
//
//
// Thanks for the fix!!!
//
//
//
//
| Mockito | /*
* Copyright (c) 2007 Mockito contributors
* This program is made available under the terms of the MIT License.
*/
package org.mockitousage.bugs;
import static org.mockito.Mockito.*;
import org.junit.Test;
import org.mockito.Mock;
import org.mockito.exceptions.verification.NeverWantedButInvoked;
import org.mockitousage.IMethods;
import org.mockitoutil.TestBase;
//see bug 138
public class VerifyingWithAnExtraCallToADifferentMockTest extends TestBase {
@Mock IMethods mock;
@Mock IMethods mockTwo;
@Test
public void shouldAllowVerifyingWhenOtherMockCallIsInTheSameLine() {
//given
when(mock.otherMethod()).thenReturn("foo");
//when
mockTwo.simpleMethod("foo");
//then
verify(mockTwo).simpleMethod(mock.otherMethod());
try {
verify(mockTwo, never()).simpleMethod(mock.otherMethod());
fail();
} catch (NeverWantedButInvoked e) {}
}
} |
||
public void testAliasing7() {
// An exported alias must preserved any referenced values in the
// referenced function.
testSame("function e(){}" +
"e.prototype['alias1'] = e.prototype.method1 = " +
"function(){this.method2()};" +
"e.prototype.method2 = function(){};");
} | com.google.javascript.jscomp.RemoveUnusedPrototypePropertiesTest::testAliasing7 | test/com/google/javascript/jscomp/RemoveUnusedPrototypePropertiesTest.java | 192 | test/com/google/javascript/jscomp/RemoveUnusedPrototypePropertiesTest.java | testAliasing7 | /*
* Copyright 2006 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
/**
* Tessts for {@link RemoveUnusedPrototypeProperties}.
*
* @author [email protected] (Nick Santos)
*/
public class RemoveUnusedPrototypePropertiesTest extends CompilerTestCase {
private static final String EXTERNS =
"IFoo.prototype.bar; var mExtern; mExtern.bExtern; mExtern['cExtern'];";
private boolean canRemoveExterns = false;
private boolean anchorUnusedVars = false;
public RemoveUnusedPrototypePropertiesTest() {
super(EXTERNS);
}
@Override
protected CompilerPass getProcessor(Compiler compiler) {
return new RemoveUnusedPrototypeProperties(compiler,
canRemoveExterns, anchorUnusedVars);
}
@Override
public void setUp() {
anchorUnusedVars = false;
canRemoveExterns = false;
}
public void testAnalyzePrototypeProperties() {
// Basic removal for prototype properties
test("function e(){}" +
"e.prototype.a = function(){};" +
"e.prototype.b = function(){};" +
"var x = new e; x.a()",
"function e(){}" +
"e.prototype.a = function(){};" +
"var x = new e; x.a()");
// Basic removal for prototype replacement
test("function e(){}" +
"e.prototype = {a: function(){}, b: function(){}};" +
"var x=new e; x.a()",
"function e(){}" +
"e.prototype = {a: function(){}};" +
"var x = new e; x.a()");
// Unused properties that were referenced in the externs file should not be
// removed
test("function e(){}" +
"e.prototype.a = function(){};" +
"e.prototype.bExtern = function(){};" +
"var x = new e;x.a()",
"function e(){}" +
"e.prototype.a = function(){};" +
"e.prototype.bExtern = function(){};" +
"var x = new e; x.a()");
test("function e(){}" +
"e.prototype = {a: function(){}, bExtern: function(){}};" +
"var x = new e; x.a()",
"function e(){}" +
"e.prototype = {a: function(){}, bExtern: function(){}};" +
"var x = new e; x.a()");
}
public void testAliasing1() {
// Aliasing a property is not enough for it to count as used
test("function e(){}" +
"e.prototype.method1 = function(){};" +
"e.prototype.method2 = function(){};" +
// aliases
"e.prototype.alias1 = e.prototype.method1;" +
"e.prototype.alias2 = e.prototype.method2;" +
"var x = new e; x.method1()",
"function e(){}" +
"e.prototype.method1 = function(){};" +
"var x = new e; x.method1()");
// Using an alias should keep it
test("function e(){}" +
"e.prototype.method1 = function(){};" +
"e.prototype.method2 = function(){};" +
// aliases
"e.prototype.alias1 = e.prototype.method1;" +
"e.prototype.alias2 = e.prototype.method2;" +
"var x=new e; x.alias1()",
"function e(){}" +
"e.prototype.method1 = function(){};" +
"e.prototype.alias1 = e.prototype.method1;" +
"var x = new e; x.alias1()");
}
public void testAliasing2() {
// Aliasing a property is not enough for it to count as used
test("function e(){}" +
"e.prototype.method1 = function(){};" +
// aliases
"e.prototype.alias1 = e.prototype.method1;" +
"(new e).method1()",
"function e(){}" +
"e.prototype.method1 = function(){};" +
"(new e).method1()");
// Using an alias should keep it
test("function e(){}" +
"e.prototype.method1 = function(){};" +
// aliases
"e.prototype.alias1 = e.prototype.method1;" +
"(new e).alias1()",
"function e(){}" +
"e.prototype.method1 = function(){};" +
"e.prototype.alias1 = e.prototype.method1;" +
"(new e).alias1()");
}
public void testAliasing3() {
// Aliasing a property is not enough for it to count as used
test("function e(){}" +
"e.prototype.method1 = function(){};" +
"e.prototype.method2 = function(){};" +
// aliases
"e.prototype['alias1'] = e.prototype.method1;" +
"e.prototype['alias2'] = e.prototype.method2;",
"function e(){}" +
"e.prototype.method1=function(){};" +
"e.prototype.method2=function(){};" +
"e.prototype[\"alias1\"]=e.prototype.method1;" +
"e.prototype[\"alias2\"]=e.prototype.method2;");
}
public void testAliasing4() {
// Aliasing a property is not enough for it to count as used
test("function e(){}" +
"e.prototype['alias1'] = e.prototype.method1 = function(){};" +
"e.prototype['alias2'] = e.prototype.method2 = function(){};",
"function e(){}" +
"e.prototype[\"alias1\"]=e.prototype.method1=function(){};" +
"e.prototype[\"alias2\"]=e.prototype.method2=function(){};");
}
public void testAliasing5() {
// An exported alias must preserved any referenced values in the
// referenced function.
test("function e(){}" +
"e.prototype.method1 = function(){this.method2()};" +
"e.prototype.method2 = function(){};" +
// aliases
"e.prototype['alias1'] = e.prototype.method1;",
"function e(){}" +
"e.prototype.method1=function(){this.method2()};" +
"e.prototype.method2=function(){};" +
"e.prototype[\"alias1\"]=e.prototype.method1;");
}
public void testAliasing6() {
// An exported alias must preserved any referenced values in the
// referenced function.
test("function e(){}" +
"e.prototype.method1 = function(){this.method2()};" +
"e.prototype.method2 = function(){};" +
// aliases
"window['alias1'] = e.prototype.method1;",
"function e(){}" +
"e.prototype.method1=function(){this.method2()};" +
"e.prototype.method2=function(){};" +
"window['alias1']=e.prototype.method1;");
}
public void testAliasing7() {
// An exported alias must preserved any referenced values in the
// referenced function.
testSame("function e(){}" +
"e.prototype['alias1'] = e.prototype.method1 = " +
"function(){this.method2()};" +
"e.prototype.method2 = function(){};");
}
public void testStatementRestriction() {
test("function e(){}" +
"var x = e.prototype.method1 = function(){};" +
"var y = new e; x()",
"function e(){}" +
"var x = e.prototype.method1 = function(){};" +
"var y = new e; x()");
}
public void testExportedMethodsByNamingConvention() {
String classAndItsMethodAliasedAsExtern =
"function Foo() {}" +
"Foo.prototype.method = function() {};" + // not removed
"Foo.prototype.unused = function() {};" + // removed
"var _externInstance = new Foo();" +
"Foo.prototype._externMethod = Foo.prototype.method"; // aliased here
String compiled =
"function Foo(){}" +
"Foo.prototype.method = function(){};" +
"var _externInstance = new Foo;" +
"Foo.prototype._externMethod = Foo.prototype.method";
test(classAndItsMethodAliasedAsExtern, compiled);
}
public void testMethodsFromExternsFileNotExported() {
canRemoveExterns = true;
String classAndItsMethodAliasedAsExtern =
"function Foo() {}" +
"Foo.prototype.bar_ = function() {};" +
"Foo.prototype.unused = function() {};" +
"var instance = new Foo;" +
"Foo.prototype.bar = Foo.prototype.bar_";
String compiled =
"function Foo(){}" +
"var instance = new Foo;";
test(classAndItsMethodAliasedAsExtern, compiled);
}
public void testExportedMethodsByNamingConventionAlwaysExported() {
canRemoveExterns = true;
String classAndItsMethodAliasedAsExtern =
"function Foo() {}" +
"Foo.prototype.method = function() {};" + // not removed
"Foo.prototype.unused = function() {};" + // removed
"var _externInstance = new Foo();" +
"Foo.prototype._externMethod = Foo.prototype.method"; // aliased here
String compiled =
"function Foo(){}" +
"Foo.prototype.method = function(){};" +
"var _externInstance = new Foo;" +
"Foo.prototype._externMethod = Foo.prototype.method";
test(classAndItsMethodAliasedAsExtern, compiled);
}
public void testExternMethodsFromExternsFile() {
String classAndItsMethodAliasedAsExtern =
"function Foo() {}" +
"Foo.prototype.bar_ = function() {};" + // not removed
"Foo.prototype.unused = function() {};" + // removed
"var instance = new Foo;" +
"Foo.prototype.bar = Foo.prototype.bar_"; // aliased here
String compiled =
"function Foo(){}" +
"Foo.prototype.bar_ = function(){};" +
"var instance = new Foo;" +
"Foo.prototype.bar = Foo.prototype.bar_";
test(classAndItsMethodAliasedAsExtern, compiled);
}
public void testPropertyReferenceGraph() {
// test a prototype property graph that looks like so:
// b -> a, c -> b, c -> a, d -> c, e -> a, e -> f
String constructor = "function Foo() {}";
String defA =
"Foo.prototype.a = function() { Foo.superClass_.a.call(this); };";
String defB = "Foo.prototype.b = function() { this.a(); };";
String defC = "Foo.prototype.c = function() { " +
"Foo.superClass_.c.call(this); this.b(); this.a(); };";
String defD = "Foo.prototype.d = function() { this.c(); };";
String defE = "Foo.prototype.e = function() { this.a(); this.f(); };";
String defF = "Foo.prototype.f = function() { };";
String fullClassDef = constructor + defA + defB + defC + defD + defE + defF;
// ensure that all prototypes are compiled out if none are used
test(fullClassDef, "");
// make sure that the right prototypes are called for each use
String callA = "(new Foo()).a();";
String callB = "(new Foo()).b();";
String callC = "(new Foo()).c();";
String callD = "(new Foo()).d();";
String callE = "(new Foo()).e();";
String callF = "(new Foo()).f();";
test(fullClassDef + callA, constructor + defA + callA);
test(fullClassDef + callB, constructor + defA + defB + callB);
test(fullClassDef + callC, constructor + defA + defB + defC + callC);
test(fullClassDef + callD, constructor + defA + defB + defC + defD + callD);
test(fullClassDef + callE, constructor + defA + defE + defF + callE);
test(fullClassDef + callF, constructor + defF + callF);
test(fullClassDef + callA + callC,
constructor + defA + defB + defC + callA + callC);
test(fullClassDef + callB + callC,
constructor + defA + defB + defC + callB + callC);
test(fullClassDef + callA + callB + callC,
constructor + defA + defB + defC + callA + callB + callC);
}
public void testPropertiesDefinedWithGetElem() {
testSame("function Foo() {} Foo.prototype['elem'] = function() {};");
testSame("function Foo() {} Foo.prototype[1 + 1] = function() {};");
}
public void testNeverRemoveImplicitlyUsedProperties() {
testSame("function Foo() {} " +
"Foo.prototype.length = 3; " +
"Foo.prototype.toString = function() { return 'Foo'; }; " +
"Foo.prototype.valueOf = function() { return 'Foo'; }; ");
}
public void testPropertyDefinedInBranch() {
test("function Foo() {} if (true) Foo.prototype.baz = function() {};",
"if (true);");
test("function Foo() {} while (true) Foo.prototype.baz = function() {};",
"while (true);");
test("function Foo() {} for (;;) Foo.prototype.baz = function() {};",
"for (;;);");
test("function Foo() {} do Foo.prototype.baz = function() {}; while(true);",
"do; while(true);");
}
public void testUsingAnonymousObjectsToDefeatRemoval() {
String constructor = "function Foo() {}";
String declaration = constructor + "Foo.prototype.baz = 3;";
test(declaration, "");
testSame(declaration + "var x = {}; x.baz = 5;");
testSame(declaration + "var x = {baz: 5};");
test(declaration + "var x = {'baz': 5};",
"var x = {'baz': 5};");
}
public void testGlobalFunctionsInGraph() {
test(
"var x = function() { (new Foo).baz(); };" +
"var y = function() { x(); };" +
"function Foo() {}" +
"Foo.prototype.baz = function() { y(); };",
"");
}
public void testGlobalFunctionsInGraph2() {
// In this example, Foo.prototype.baz is a global reference to
// Foo, and Foo has a reference to baz. So everything stays in.
// TODO(nicksantos): We should be able to make the graph more fine-grained
// here. Instead of Foo.prototype.bar creating a global reference to Foo,
// it should create a reference from .bar to Foo. That will let us
// compile this away to nothing.
testSame(
"var x = function() { (new Foo).baz(); };" +
"var y = function() { x(); };" +
"function Foo() { this.baz(); }" +
"Foo.prototype.baz = function() { y(); };");
}
public void testGlobalFunctionsInGraph3() {
test(
"var x = function() { (new Foo).baz(); };" +
"var y = function() { x(); };" +
"function Foo() { this.baz(); }" +
"Foo.prototype.baz = function() { x(); };",
"var x = function() { (new Foo).baz(); };" +
"function Foo() { this.baz(); }" +
"Foo.prototype.baz = function() { x(); };");
}
public void testGlobalFunctionsInGraph4() {
test(
"var x = function() { (new Foo).baz(); };" +
"var y = function() { x(); };" +
"function Foo() { Foo.prototype.baz = function() { y(); }; }",
"");
}
public void testGlobalFunctionsInGraph5() {
test(
"function Foo() {}" +
"Foo.prototype.methodA = function() {};" +
"function x() { (new Foo).methodA(); }" +
"Foo.prototype.methodB = function() { x(); };",
"");
anchorUnusedVars = true;
test(
"function Foo() {}" +
"Foo.prototype.methodA = function() {};" +
"function x() { (new Foo).methodA(); }" +
"Foo.prototype.methodB = function() { x(); };",
"function Foo() {}" +
"Foo.prototype.methodA = function() {};" +
"function x() { (new Foo).methodA(); }");
}
public void testGlobalFunctionsInGraph6() {
testSame(
"function Foo() {}" +
"Foo.prototype.methodA = function() {};" +
"function x() { (new Foo).methodA(); }" +
"Foo.prototype.methodB = function() { x(); };" +
"(new Foo).methodB();");
}
public void testGlobalFunctionsInGraph7() {
testSame(
"function Foo() {}" +
"Foo.prototype.methodA = function() {};" +
"this.methodA();");
}
public void testGetterBaseline() {
anchorUnusedVars = true;
test(
"function Foo() {}" +
"Foo.prototype = { " +
" methodA: function() {}," +
" methodB: function() { x(); }" +
"};" +
"function x() { (new Foo).methodA(); }",
"function Foo() {}" +
"Foo.prototype = { " +
" methodA: function() {}" +
"};" +
"function x() { (new Foo).methodA(); }");
}
public void testGetter1() {
test(
"function Foo() {}" +
"Foo.prototype = { " +
" get methodA() {}," +
" get methodB() { x(); }" +
"};" +
"function x() { (new Foo).methodA; }",
"function Foo() {}" +
"Foo.prototype = {};");
anchorUnusedVars = true;
test(
"function Foo() {}" +
"Foo.prototype = { " +
" get methodA() {}," +
" get methodB() { x(); }" +
"};" +
"function x() { (new Foo).methodA; }",
"function Foo() {}" +
"Foo.prototype = { " +
" get methodA() {}" +
"};" +
"function x() { (new Foo).methodA; }");
}
public void testGetter2() {
anchorUnusedVars = true;
test(
"function Foo() {}" +
"Foo.prototype = { " +
" get methodA() {}," +
" set methodA(a) {}," +
" get methodB() { x(); }," +
" set methodB(a) { x(); }" +
"};" +
"function x() { (new Foo).methodA; }",
"function Foo() {}" +
"Foo.prototype = { " +
" get methodA() {}," +
" set methodA(a) {}" +
"};" +
"function x() { (new Foo).methodA; }");
}
} | // You are a professional Java test case writer, please create a test case named `testAliasing7` for the issue `Closure-459`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-459
//
// ## Issue-Title:
// Advanced compilations renames a function and then deletes it, leaving a reference to a renamed but non-existent function
//
// ## Issue-Description:
// If we provide the below code to advanced:
//
//
// function A() {
// this.\_x = 1;
// }
//
// A.prototype['func1'] = // done to save public reference to func1
// A.prototype.func1 = function() {
// this.\_x = 2;
// this.func2();
// }
//
// A.prototype.func2 = function() {
// this.\_x = 3;
// this.func3();
// }
//
// window['A'] = A;
//
//
// We get the output:
//
//
// function a() {
// this.a = 1
// }
// a.prototype.func1 = a.prototype.b = function() {
// this.a = 2;
// this.c() // Problem!
// };
// window.A = a;
//
//
// So the compiler emits no errors, and renames 'func2' to 'c' but ends up throwing away the definition of that function!
//
// The problem arises when I use:
//
// A.prototype['func1'] = // done to save public reference to func1
// A.prototype.func1 = function() {
// ...
// }
//
// The ['func1'] line is apparently enough to save the reference correctly, but also has the side effect of causing the function innards to do the wrong thing.
//
// I can of course instead write it as:
//
// A.prototype['func1'] = A.prototype.func1;
// A.prototype.func1 = function() {
// this.\_x = 2;
// this.func2();
// }
//
// In which case Advanced will compile correctly and the results will also be valid.
//
// function a() {
// this.a = 1
// }
// a.prototype.func1 = a.prototype.b;
// a.prototype.b = function() {
// this.a = 2;
// this.a = 3 // func2, correctly minified
// };
// window.A = a;
//
//
// For now I can just use the expected way of declaring that func1 export, but since the compiler returns with no errors or warnings and creates a function with no definition, it seems worth reporting.
//
//
public void testAliasing7() {
| 192 | 67 | 185 | test/com/google/javascript/jscomp/RemoveUnusedPrototypePropertiesTest.java | test | ```markdown
## Issue-ID: Closure-459
## Issue-Title:
Advanced compilations renames a function and then deletes it, leaving a reference to a renamed but non-existent function
## Issue-Description:
If we provide the below code to advanced:
function A() {
this.\_x = 1;
}
A.prototype['func1'] = // done to save public reference to func1
A.prototype.func1 = function() {
this.\_x = 2;
this.func2();
}
A.prototype.func2 = function() {
this.\_x = 3;
this.func3();
}
window['A'] = A;
We get the output:
function a() {
this.a = 1
}
a.prototype.func1 = a.prototype.b = function() {
this.a = 2;
this.c() // Problem!
};
window.A = a;
So the compiler emits no errors, and renames 'func2' to 'c' but ends up throwing away the definition of that function!
The problem arises when I use:
A.prototype['func1'] = // done to save public reference to func1
A.prototype.func1 = function() {
...
}
The ['func1'] line is apparently enough to save the reference correctly, but also has the side effect of causing the function innards to do the wrong thing.
I can of course instead write it as:
A.prototype['func1'] = A.prototype.func1;
A.prototype.func1 = function() {
this.\_x = 2;
this.func2();
}
In which case Advanced will compile correctly and the results will also be valid.
function a() {
this.a = 1
}
a.prototype.func1 = a.prototype.b;
a.prototype.b = function() {
this.a = 2;
this.a = 3 // func2, correctly minified
};
window.A = a;
For now I can just use the expected way of declaring that func1 export, but since the compiler returns with no errors or warnings and creates a function with no definition, it seems worth reporting.
```
You are a professional Java test case writer, please create a test case named `testAliasing7` for the issue `Closure-459`, utilizing the provided issue report information and the following function signature.
```java
public void testAliasing7() {
```
| 185 | [
"com.google.javascript.jscomp.AnalyzePrototypeProperties"
] | 97934d75b93db81f6b0c8c4eb849fa5e05b3c08979b53eec796dea72a00ae04f | public void testAliasing7() | // You are a professional Java test case writer, please create a test case named `testAliasing7` for the issue `Closure-459`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-459
//
// ## Issue-Title:
// Advanced compilations renames a function and then deletes it, leaving a reference to a renamed but non-existent function
//
// ## Issue-Description:
// If we provide the below code to advanced:
//
//
// function A() {
// this.\_x = 1;
// }
//
// A.prototype['func1'] = // done to save public reference to func1
// A.prototype.func1 = function() {
// this.\_x = 2;
// this.func2();
// }
//
// A.prototype.func2 = function() {
// this.\_x = 3;
// this.func3();
// }
//
// window['A'] = A;
//
//
// We get the output:
//
//
// function a() {
// this.a = 1
// }
// a.prototype.func1 = a.prototype.b = function() {
// this.a = 2;
// this.c() // Problem!
// };
// window.A = a;
//
//
// So the compiler emits no errors, and renames 'func2' to 'c' but ends up throwing away the definition of that function!
//
// The problem arises when I use:
//
// A.prototype['func1'] = // done to save public reference to func1
// A.prototype.func1 = function() {
// ...
// }
//
// The ['func1'] line is apparently enough to save the reference correctly, but also has the side effect of causing the function innards to do the wrong thing.
//
// I can of course instead write it as:
//
// A.prototype['func1'] = A.prototype.func1;
// A.prototype.func1 = function() {
// this.\_x = 2;
// this.func2();
// }
//
// In which case Advanced will compile correctly and the results will also be valid.
//
// function a() {
// this.a = 1
// }
// a.prototype.func1 = a.prototype.b;
// a.prototype.b = function() {
// this.a = 2;
// this.a = 3 // func2, correctly minified
// };
// window.A = a;
//
//
// For now I can just use the expected way of declaring that func1 export, but since the compiler returns with no errors or warnings and creates a function with no definition, it seems worth reporting.
//
//
| Closure | /*
* Copyright 2006 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
/**
* Tessts for {@link RemoveUnusedPrototypeProperties}.
*
* @author [email protected] (Nick Santos)
*/
public class RemoveUnusedPrototypePropertiesTest extends CompilerTestCase {
private static final String EXTERNS =
"IFoo.prototype.bar; var mExtern; mExtern.bExtern; mExtern['cExtern'];";
private boolean canRemoveExterns = false;
private boolean anchorUnusedVars = false;
public RemoveUnusedPrototypePropertiesTest() {
super(EXTERNS);
}
@Override
protected CompilerPass getProcessor(Compiler compiler) {
return new RemoveUnusedPrototypeProperties(compiler,
canRemoveExterns, anchorUnusedVars);
}
@Override
public void setUp() {
anchorUnusedVars = false;
canRemoveExterns = false;
}
public void testAnalyzePrototypeProperties() {
// Basic removal for prototype properties
test("function e(){}" +
"e.prototype.a = function(){};" +
"e.prototype.b = function(){};" +
"var x = new e; x.a()",
"function e(){}" +
"e.prototype.a = function(){};" +
"var x = new e; x.a()");
// Basic removal for prototype replacement
test("function e(){}" +
"e.prototype = {a: function(){}, b: function(){}};" +
"var x=new e; x.a()",
"function e(){}" +
"e.prototype = {a: function(){}};" +
"var x = new e; x.a()");
// Unused properties that were referenced in the externs file should not be
// removed
test("function e(){}" +
"e.prototype.a = function(){};" +
"e.prototype.bExtern = function(){};" +
"var x = new e;x.a()",
"function e(){}" +
"e.prototype.a = function(){};" +
"e.prototype.bExtern = function(){};" +
"var x = new e; x.a()");
test("function e(){}" +
"e.prototype = {a: function(){}, bExtern: function(){}};" +
"var x = new e; x.a()",
"function e(){}" +
"e.prototype = {a: function(){}, bExtern: function(){}};" +
"var x = new e; x.a()");
}
public void testAliasing1() {
// Aliasing a property is not enough for it to count as used
test("function e(){}" +
"e.prototype.method1 = function(){};" +
"e.prototype.method2 = function(){};" +
// aliases
"e.prototype.alias1 = e.prototype.method1;" +
"e.prototype.alias2 = e.prototype.method2;" +
"var x = new e; x.method1()",
"function e(){}" +
"e.prototype.method1 = function(){};" +
"var x = new e; x.method1()");
// Using an alias should keep it
test("function e(){}" +
"e.prototype.method1 = function(){};" +
"e.prototype.method2 = function(){};" +
// aliases
"e.prototype.alias1 = e.prototype.method1;" +
"e.prototype.alias2 = e.prototype.method2;" +
"var x=new e; x.alias1()",
"function e(){}" +
"e.prototype.method1 = function(){};" +
"e.prototype.alias1 = e.prototype.method1;" +
"var x = new e; x.alias1()");
}
public void testAliasing2() {
// Aliasing a property is not enough for it to count as used
test("function e(){}" +
"e.prototype.method1 = function(){};" +
// aliases
"e.prototype.alias1 = e.prototype.method1;" +
"(new e).method1()",
"function e(){}" +
"e.prototype.method1 = function(){};" +
"(new e).method1()");
// Using an alias should keep it
test("function e(){}" +
"e.prototype.method1 = function(){};" +
// aliases
"e.prototype.alias1 = e.prototype.method1;" +
"(new e).alias1()",
"function e(){}" +
"e.prototype.method1 = function(){};" +
"e.prototype.alias1 = e.prototype.method1;" +
"(new e).alias1()");
}
public void testAliasing3() {
// Aliasing a property is not enough for it to count as used
test("function e(){}" +
"e.prototype.method1 = function(){};" +
"e.prototype.method2 = function(){};" +
// aliases
"e.prototype['alias1'] = e.prototype.method1;" +
"e.prototype['alias2'] = e.prototype.method2;",
"function e(){}" +
"e.prototype.method1=function(){};" +
"e.prototype.method2=function(){};" +
"e.prototype[\"alias1\"]=e.prototype.method1;" +
"e.prototype[\"alias2\"]=e.prototype.method2;");
}
public void testAliasing4() {
// Aliasing a property is not enough for it to count as used
test("function e(){}" +
"e.prototype['alias1'] = e.prototype.method1 = function(){};" +
"e.prototype['alias2'] = e.prototype.method2 = function(){};",
"function e(){}" +
"e.prototype[\"alias1\"]=e.prototype.method1=function(){};" +
"e.prototype[\"alias2\"]=e.prototype.method2=function(){};");
}
public void testAliasing5() {
// An exported alias must preserved any referenced values in the
// referenced function.
test("function e(){}" +
"e.prototype.method1 = function(){this.method2()};" +
"e.prototype.method2 = function(){};" +
// aliases
"e.prototype['alias1'] = e.prototype.method1;",
"function e(){}" +
"e.prototype.method1=function(){this.method2()};" +
"e.prototype.method2=function(){};" +
"e.prototype[\"alias1\"]=e.prototype.method1;");
}
public void testAliasing6() {
// An exported alias must preserved any referenced values in the
// referenced function.
test("function e(){}" +
"e.prototype.method1 = function(){this.method2()};" +
"e.prototype.method2 = function(){};" +
// aliases
"window['alias1'] = e.prototype.method1;",
"function e(){}" +
"e.prototype.method1=function(){this.method2()};" +
"e.prototype.method2=function(){};" +
"window['alias1']=e.prototype.method1;");
}
public void testAliasing7() {
// An exported alias must preserved any referenced values in the
// referenced function.
testSame("function e(){}" +
"e.prototype['alias1'] = e.prototype.method1 = " +
"function(){this.method2()};" +
"e.prototype.method2 = function(){};");
}
public void testStatementRestriction() {
test("function e(){}" +
"var x = e.prototype.method1 = function(){};" +
"var y = new e; x()",
"function e(){}" +
"var x = e.prototype.method1 = function(){};" +
"var y = new e; x()");
}
public void testExportedMethodsByNamingConvention() {
String classAndItsMethodAliasedAsExtern =
"function Foo() {}" +
"Foo.prototype.method = function() {};" + // not removed
"Foo.prototype.unused = function() {};" + // removed
"var _externInstance = new Foo();" +
"Foo.prototype._externMethod = Foo.prototype.method"; // aliased here
String compiled =
"function Foo(){}" +
"Foo.prototype.method = function(){};" +
"var _externInstance = new Foo;" +
"Foo.prototype._externMethod = Foo.prototype.method";
test(classAndItsMethodAliasedAsExtern, compiled);
}
public void testMethodsFromExternsFileNotExported() {
canRemoveExterns = true;
String classAndItsMethodAliasedAsExtern =
"function Foo() {}" +
"Foo.prototype.bar_ = function() {};" +
"Foo.prototype.unused = function() {};" +
"var instance = new Foo;" +
"Foo.prototype.bar = Foo.prototype.bar_";
String compiled =
"function Foo(){}" +
"var instance = new Foo;";
test(classAndItsMethodAliasedAsExtern, compiled);
}
public void testExportedMethodsByNamingConventionAlwaysExported() {
canRemoveExterns = true;
String classAndItsMethodAliasedAsExtern =
"function Foo() {}" +
"Foo.prototype.method = function() {};" + // not removed
"Foo.prototype.unused = function() {};" + // removed
"var _externInstance = new Foo();" +
"Foo.prototype._externMethod = Foo.prototype.method"; // aliased here
String compiled =
"function Foo(){}" +
"Foo.prototype.method = function(){};" +
"var _externInstance = new Foo;" +
"Foo.prototype._externMethod = Foo.prototype.method";
test(classAndItsMethodAliasedAsExtern, compiled);
}
public void testExternMethodsFromExternsFile() {
String classAndItsMethodAliasedAsExtern =
"function Foo() {}" +
"Foo.prototype.bar_ = function() {};" + // not removed
"Foo.prototype.unused = function() {};" + // removed
"var instance = new Foo;" +
"Foo.prototype.bar = Foo.prototype.bar_"; // aliased here
String compiled =
"function Foo(){}" +
"Foo.prototype.bar_ = function(){};" +
"var instance = new Foo;" +
"Foo.prototype.bar = Foo.prototype.bar_";
test(classAndItsMethodAliasedAsExtern, compiled);
}
public void testPropertyReferenceGraph() {
// test a prototype property graph that looks like so:
// b -> a, c -> b, c -> a, d -> c, e -> a, e -> f
String constructor = "function Foo() {}";
String defA =
"Foo.prototype.a = function() { Foo.superClass_.a.call(this); };";
String defB = "Foo.prototype.b = function() { this.a(); };";
String defC = "Foo.prototype.c = function() { " +
"Foo.superClass_.c.call(this); this.b(); this.a(); };";
String defD = "Foo.prototype.d = function() { this.c(); };";
String defE = "Foo.prototype.e = function() { this.a(); this.f(); };";
String defF = "Foo.prototype.f = function() { };";
String fullClassDef = constructor + defA + defB + defC + defD + defE + defF;
// ensure that all prototypes are compiled out if none are used
test(fullClassDef, "");
// make sure that the right prototypes are called for each use
String callA = "(new Foo()).a();";
String callB = "(new Foo()).b();";
String callC = "(new Foo()).c();";
String callD = "(new Foo()).d();";
String callE = "(new Foo()).e();";
String callF = "(new Foo()).f();";
test(fullClassDef + callA, constructor + defA + callA);
test(fullClassDef + callB, constructor + defA + defB + callB);
test(fullClassDef + callC, constructor + defA + defB + defC + callC);
test(fullClassDef + callD, constructor + defA + defB + defC + defD + callD);
test(fullClassDef + callE, constructor + defA + defE + defF + callE);
test(fullClassDef + callF, constructor + defF + callF);
test(fullClassDef + callA + callC,
constructor + defA + defB + defC + callA + callC);
test(fullClassDef + callB + callC,
constructor + defA + defB + defC + callB + callC);
test(fullClassDef + callA + callB + callC,
constructor + defA + defB + defC + callA + callB + callC);
}
public void testPropertiesDefinedWithGetElem() {
testSame("function Foo() {} Foo.prototype['elem'] = function() {};");
testSame("function Foo() {} Foo.prototype[1 + 1] = function() {};");
}
public void testNeverRemoveImplicitlyUsedProperties() {
testSame("function Foo() {} " +
"Foo.prototype.length = 3; " +
"Foo.prototype.toString = function() { return 'Foo'; }; " +
"Foo.prototype.valueOf = function() { return 'Foo'; }; ");
}
public void testPropertyDefinedInBranch() {
test("function Foo() {} if (true) Foo.prototype.baz = function() {};",
"if (true);");
test("function Foo() {} while (true) Foo.prototype.baz = function() {};",
"while (true);");
test("function Foo() {} for (;;) Foo.prototype.baz = function() {};",
"for (;;);");
test("function Foo() {} do Foo.prototype.baz = function() {}; while(true);",
"do; while(true);");
}
public void testUsingAnonymousObjectsToDefeatRemoval() {
String constructor = "function Foo() {}";
String declaration = constructor + "Foo.prototype.baz = 3;";
test(declaration, "");
testSame(declaration + "var x = {}; x.baz = 5;");
testSame(declaration + "var x = {baz: 5};");
test(declaration + "var x = {'baz': 5};",
"var x = {'baz': 5};");
}
public void testGlobalFunctionsInGraph() {
test(
"var x = function() { (new Foo).baz(); };" +
"var y = function() { x(); };" +
"function Foo() {}" +
"Foo.prototype.baz = function() { y(); };",
"");
}
public void testGlobalFunctionsInGraph2() {
// In this example, Foo.prototype.baz is a global reference to
// Foo, and Foo has a reference to baz. So everything stays in.
// TODO(nicksantos): We should be able to make the graph more fine-grained
// here. Instead of Foo.prototype.bar creating a global reference to Foo,
// it should create a reference from .bar to Foo. That will let us
// compile this away to nothing.
testSame(
"var x = function() { (new Foo).baz(); };" +
"var y = function() { x(); };" +
"function Foo() { this.baz(); }" +
"Foo.prototype.baz = function() { y(); };");
}
public void testGlobalFunctionsInGraph3() {
test(
"var x = function() { (new Foo).baz(); };" +
"var y = function() { x(); };" +
"function Foo() { this.baz(); }" +
"Foo.prototype.baz = function() { x(); };",
"var x = function() { (new Foo).baz(); };" +
"function Foo() { this.baz(); }" +
"Foo.prototype.baz = function() { x(); };");
}
public void testGlobalFunctionsInGraph4() {
test(
"var x = function() { (new Foo).baz(); };" +
"var y = function() { x(); };" +
"function Foo() { Foo.prototype.baz = function() { y(); }; }",
"");
}
public void testGlobalFunctionsInGraph5() {
test(
"function Foo() {}" +
"Foo.prototype.methodA = function() {};" +
"function x() { (new Foo).methodA(); }" +
"Foo.prototype.methodB = function() { x(); };",
"");
anchorUnusedVars = true;
test(
"function Foo() {}" +
"Foo.prototype.methodA = function() {};" +
"function x() { (new Foo).methodA(); }" +
"Foo.prototype.methodB = function() { x(); };",
"function Foo() {}" +
"Foo.prototype.methodA = function() {};" +
"function x() { (new Foo).methodA(); }");
}
public void testGlobalFunctionsInGraph6() {
testSame(
"function Foo() {}" +
"Foo.prototype.methodA = function() {};" +
"function x() { (new Foo).methodA(); }" +
"Foo.prototype.methodB = function() { x(); };" +
"(new Foo).methodB();");
}
public void testGlobalFunctionsInGraph7() {
testSame(
"function Foo() {}" +
"Foo.prototype.methodA = function() {};" +
"this.methodA();");
}
public void testGetterBaseline() {
anchorUnusedVars = true;
test(
"function Foo() {}" +
"Foo.prototype = { " +
" methodA: function() {}," +
" methodB: function() { x(); }" +
"};" +
"function x() { (new Foo).methodA(); }",
"function Foo() {}" +
"Foo.prototype = { " +
" methodA: function() {}" +
"};" +
"function x() { (new Foo).methodA(); }");
}
public void testGetter1() {
test(
"function Foo() {}" +
"Foo.prototype = { " +
" get methodA() {}," +
" get methodB() { x(); }" +
"};" +
"function x() { (new Foo).methodA; }",
"function Foo() {}" +
"Foo.prototype = {};");
anchorUnusedVars = true;
test(
"function Foo() {}" +
"Foo.prototype = { " +
" get methodA() {}," +
" get methodB() { x(); }" +
"};" +
"function x() { (new Foo).methodA; }",
"function Foo() {}" +
"Foo.prototype = { " +
" get methodA() {}" +
"};" +
"function x() { (new Foo).methodA; }");
}
public void testGetter2() {
anchorUnusedVars = true;
test(
"function Foo() {}" +
"Foo.prototype = { " +
" get methodA() {}," +
" set methodA(a) {}," +
" get methodB() { x(); }," +
" set methodB(a) { x(); }" +
"};" +
"function x() { (new Foo).methodA; }",
"function Foo() {}" +
"Foo.prototype = { " +
" get methodA() {}," +
" set methodA(a) {}" +
"};" +
"function x() { (new Foo).methodA; }");
}
} |
||
@Test
public void should_return_empty_iterable() throws Exception {
assertFalse(((Iterable) values.returnValueFor(Iterable.class)).iterator().hasNext());
} | org.mockito.internal.stubbing.defaultanswers.ReturnsEmptyValuesTest::should_return_empty_iterable | test/org/mockito/internal/stubbing/defaultanswers/ReturnsEmptyValuesTest.java | 57 | test/org/mockito/internal/stubbing/defaultanswers/ReturnsEmptyValuesTest.java | should_return_empty_iterable | /*
* Copyright (c) 2007 Mockito contributors
* This program is made available under the terms of the MIT License.
*/
package org.mockito.internal.stubbing.defaultanswers;
import static org.mockito.Mockito.mock;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Date;
import java.util.HashMap;
import java.util.HashSet;
import java.util.LinkedHashMap;
import java.util.LinkedHashSet;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.SortedMap;
import java.util.SortedSet;
import java.util.TreeMap;
import java.util.TreeSet;
import org.junit.Test;
import org.mockito.invocation.Invocation;
import org.mockitoutil.TestBase;
@SuppressWarnings("unchecked")
public class ReturnsEmptyValuesTest extends TestBase {
ReturnsEmptyValues values = new ReturnsEmptyValues();
@Test public void should_return_empty_collections_or_null_for_non_collections() {
assertTrue(((Collection) values.returnValueFor(Collection.class)).isEmpty());
assertTrue(((Set) values.returnValueFor(Set.class)).isEmpty());
assertTrue(((SortedSet) values.returnValueFor(SortedSet.class)).isEmpty());
assertTrue(((HashSet) values.returnValueFor(HashSet.class)).isEmpty());
assertTrue(((TreeSet) values.returnValueFor(TreeSet.class)).isEmpty());
assertTrue(((LinkedHashSet) values.returnValueFor(LinkedHashSet.class)).isEmpty());
assertTrue(((List) values.returnValueFor(List.class)).isEmpty());
assertTrue(((ArrayList) values.returnValueFor(ArrayList.class)).isEmpty());
assertTrue(((LinkedList) values.returnValueFor(LinkedList.class)).isEmpty());
assertTrue(((Map) values.returnValueFor(Map.class)).isEmpty());
assertTrue(((SortedMap) values.returnValueFor(SortedMap.class)).isEmpty());
assertTrue(((HashMap) values.returnValueFor(HashMap.class)).isEmpty());
assertTrue(((TreeMap) values.returnValueFor(TreeMap.class)).isEmpty());
assertTrue(((LinkedHashMap) values.returnValueFor(LinkedHashMap.class)).isEmpty());
assertNull(values.returnValueFor(String.class));
}
@Test
public void should_return_empty_iterable() throws Exception {
assertFalse(((Iterable) values.returnValueFor(Iterable.class)).iterator().hasNext());
}
@Test public void should_return_primitive() {
assertEquals(false, values.returnValueFor(Boolean.TYPE));
assertEquals((char) 0, values.returnValueFor(Character.TYPE));
assertEquals((byte) 0, values.returnValueFor(Byte.TYPE));
assertEquals((short) 0, values.returnValueFor(Short.TYPE));
assertEquals(0, values.returnValueFor(Integer.TYPE));
assertEquals(0L, values.returnValueFor(Long.TYPE));
assertEquals(0F, values.returnValueFor(Float.TYPE));
assertEquals(0D, values.returnValueFor(Double.TYPE));
}
@Test public void should_return_non_zero_for_compareTo_method() {
//given
Date d = mock(Date.class);
d.compareTo(new Date());
Invocation compareTo = this.getLastInvocation();
//when
Object result = values.answer(compareTo);
//then
assertTrue(result != (Object) 0);
}
@Test public void should_return_zero_if_mock_is_compared_to_itself() {
//given
Date d = mock(Date.class);
d.compareTo(d);
Invocation compareTo = this.getLastInvocation();
//when
Object result = values.answer(compareTo);
//then
assertEquals(0, result);
}
} | // You are a professional Java test case writer, please create a test case named `should_return_empty_iterable` for the issue `Mockito-210`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Mockito-210
//
// ## Issue-Title:
// Return empty value for Iterables
//
// ## Issue-Description:
// <http://code.google.com/p/mockito/issues/detail?id=175>
//
//
// I expect an Iterable to be mocked by default with an empty Iterable. I understand from the initial issue this behavior would be introduced in Mockito 2, but beta-8 still returns null.
//
//
// Could we return null for Iterables ?
//
//
// Should we have the same behavior for Iterator ?
//
//
// Thanks
//
//
//
//
@Test
public void should_return_empty_iterable() throws Exception {
| 57 | 18 | 54 | test/org/mockito/internal/stubbing/defaultanswers/ReturnsEmptyValuesTest.java | test | ```markdown
## Issue-ID: Mockito-210
## Issue-Title:
Return empty value for Iterables
## Issue-Description:
<http://code.google.com/p/mockito/issues/detail?id=175>
I expect an Iterable to be mocked by default with an empty Iterable. I understand from the initial issue this behavior would be introduced in Mockito 2, but beta-8 still returns null.
Could we return null for Iterables ?
Should we have the same behavior for Iterator ?
Thanks
```
You are a professional Java test case writer, please create a test case named `should_return_empty_iterable` for the issue `Mockito-210`, utilizing the provided issue report information and the following function signature.
```java
@Test
public void should_return_empty_iterable() throws Exception {
```
| 54 | [
"org.mockito.internal.stubbing.defaultanswers.ReturnsEmptyValues"
] | 992b09a019da11e55005c6a755c34f2507a8a6ea7d2655c4cc23681dc57f3e25 | @Test
public void should_return_empty_iterable() throws Exception | // You are a professional Java test case writer, please create a test case named `should_return_empty_iterable` for the issue `Mockito-210`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Mockito-210
//
// ## Issue-Title:
// Return empty value for Iterables
//
// ## Issue-Description:
// <http://code.google.com/p/mockito/issues/detail?id=175>
//
//
// I expect an Iterable to be mocked by default with an empty Iterable. I understand from the initial issue this behavior would be introduced in Mockito 2, but beta-8 still returns null.
//
//
// Could we return null for Iterables ?
//
//
// Should we have the same behavior for Iterator ?
//
//
// Thanks
//
//
//
//
| Mockito | /*
* Copyright (c) 2007 Mockito contributors
* This program is made available under the terms of the MIT License.
*/
package org.mockito.internal.stubbing.defaultanswers;
import static org.mockito.Mockito.mock;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Date;
import java.util.HashMap;
import java.util.HashSet;
import java.util.LinkedHashMap;
import java.util.LinkedHashSet;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.SortedMap;
import java.util.SortedSet;
import java.util.TreeMap;
import java.util.TreeSet;
import org.junit.Test;
import org.mockito.invocation.Invocation;
import org.mockitoutil.TestBase;
@SuppressWarnings("unchecked")
public class ReturnsEmptyValuesTest extends TestBase {
ReturnsEmptyValues values = new ReturnsEmptyValues();
@Test public void should_return_empty_collections_or_null_for_non_collections() {
assertTrue(((Collection) values.returnValueFor(Collection.class)).isEmpty());
assertTrue(((Set) values.returnValueFor(Set.class)).isEmpty());
assertTrue(((SortedSet) values.returnValueFor(SortedSet.class)).isEmpty());
assertTrue(((HashSet) values.returnValueFor(HashSet.class)).isEmpty());
assertTrue(((TreeSet) values.returnValueFor(TreeSet.class)).isEmpty());
assertTrue(((LinkedHashSet) values.returnValueFor(LinkedHashSet.class)).isEmpty());
assertTrue(((List) values.returnValueFor(List.class)).isEmpty());
assertTrue(((ArrayList) values.returnValueFor(ArrayList.class)).isEmpty());
assertTrue(((LinkedList) values.returnValueFor(LinkedList.class)).isEmpty());
assertTrue(((Map) values.returnValueFor(Map.class)).isEmpty());
assertTrue(((SortedMap) values.returnValueFor(SortedMap.class)).isEmpty());
assertTrue(((HashMap) values.returnValueFor(HashMap.class)).isEmpty());
assertTrue(((TreeMap) values.returnValueFor(TreeMap.class)).isEmpty());
assertTrue(((LinkedHashMap) values.returnValueFor(LinkedHashMap.class)).isEmpty());
assertNull(values.returnValueFor(String.class));
}
@Test
public void should_return_empty_iterable() throws Exception {
assertFalse(((Iterable) values.returnValueFor(Iterable.class)).iterator().hasNext());
}
@Test public void should_return_primitive() {
assertEquals(false, values.returnValueFor(Boolean.TYPE));
assertEquals((char) 0, values.returnValueFor(Character.TYPE));
assertEquals((byte) 0, values.returnValueFor(Byte.TYPE));
assertEquals((short) 0, values.returnValueFor(Short.TYPE));
assertEquals(0, values.returnValueFor(Integer.TYPE));
assertEquals(0L, values.returnValueFor(Long.TYPE));
assertEquals(0F, values.returnValueFor(Float.TYPE));
assertEquals(0D, values.returnValueFor(Double.TYPE));
}
@Test public void should_return_non_zero_for_compareTo_method() {
//given
Date d = mock(Date.class);
d.compareTo(new Date());
Invocation compareTo = this.getLastInvocation();
//when
Object result = values.answer(compareTo);
//then
assertTrue(result != (Object) 0);
}
@Test public void should_return_zero_if_mock_is_compared_to_itself() {
//given
Date d = mock(Date.class);
d.compareTo(d);
Invocation compareTo = this.getLastInvocation();
//when
Object result = values.answer(compareTo);
//then
assertEquals(0, result);
}
} |
||
public void testToPeriod_fixedZone() throws Throwable {
DateTimeZone zone = DateTimeZone.getDefault();
try {
DateTimeZone.setDefault(DateTimeZone.forOffsetHours(2));
long length =
(4L + (3L * 7L) + (2L * 30L) + 365L) * DateTimeConstants.MILLIS_PER_DAY +
5L * DateTimeConstants.MILLIS_PER_HOUR +
6L * DateTimeConstants.MILLIS_PER_MINUTE +
7L * DateTimeConstants.MILLIS_PER_SECOND + 8L;
Duration dur = new Duration(length);
Period test = dur.toPeriod();
assertEquals(0, test.getYears()); // (4 + (3 * 7) + (2 * 30) + 365) == 450
assertEquals(0, test.getMonths());
assertEquals(0, test.getWeeks());
assertEquals(0, test.getDays());
assertEquals((450 * 24) + 5, test.getHours());
assertEquals(6, test.getMinutes());
assertEquals(7, test.getSeconds());
assertEquals(8, test.getMillis());
} finally {
DateTimeZone.setDefault(zone);
}
} | org.joda.time.TestDuration_Basics::testToPeriod_fixedZone | src/test/java/org/joda/time/TestDuration_Basics.java | 491 | src/test/java/org/joda/time/TestDuration_Basics.java | testToPeriod_fixedZone | /*
* Copyright 2001-2009 Stephen Colebourne
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.joda.time;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.util.Locale;
import java.util.TimeZone;
import junit.framework.TestCase;
import junit.framework.TestSuite;
import org.joda.time.base.AbstractDuration;
import org.joda.time.base.BaseDuration;
import org.joda.time.chrono.ISOChronology;
/**
* This class is a Junit unit test for Duration.
*
* @author Stephen Colebourne
*/
public class TestDuration_Basics extends TestCase {
// Test in 2002/03 as time zones are more well known
// (before the late 90's they were all over the place)
private static final DateTimeZone LONDON = DateTimeZone.forID("Europe/London");
long y2002days = 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 +
365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365;
long y2003days = 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 +
365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365 + 365;
// 2002-06-09
private long TEST_TIME_NOW =
(y2002days + 31L + 28L + 31L + 30L + 31L + 9L -1L) * DateTimeConstants.MILLIS_PER_DAY;
// 2002-04-05
private long TEST_TIME1 =
(y2002days + 31L + 28L + 31L + 5L -1L) * DateTimeConstants.MILLIS_PER_DAY
+ 12L * DateTimeConstants.MILLIS_PER_HOUR
+ 24L * DateTimeConstants.MILLIS_PER_MINUTE;
// 2003-05-06
private long TEST_TIME2 =
(y2003days + 31L + 28L + 31L + 30L + 6L -1L) * DateTimeConstants.MILLIS_PER_DAY
+ 14L * DateTimeConstants.MILLIS_PER_HOUR
+ 28L * DateTimeConstants.MILLIS_PER_MINUTE;
private DateTimeZone originalDateTimeZone = null;
private TimeZone originalTimeZone = null;
private Locale originalLocale = null;
public static void main(String[] args) {
junit.textui.TestRunner.run(suite());
}
public static TestSuite suite() {
return new TestSuite(TestDuration_Basics.class);
}
public TestDuration_Basics(String name) {
super(name);
}
protected void setUp() throws Exception {
DateTimeUtils.setCurrentMillisFixed(TEST_TIME_NOW);
originalDateTimeZone = DateTimeZone.getDefault();
originalTimeZone = TimeZone.getDefault();
originalLocale = Locale.getDefault();
DateTimeZone.setDefault(LONDON);
TimeZone.setDefault(TimeZone.getTimeZone("Europe/London"));
Locale.setDefault(Locale.UK);
}
protected void tearDown() throws Exception {
DateTimeUtils.setCurrentMillisSystem();
DateTimeZone.setDefault(originalDateTimeZone);
TimeZone.setDefault(originalTimeZone);
Locale.setDefault(originalLocale);
originalDateTimeZone = null;
originalTimeZone = null;
originalLocale = null;
}
//-----------------------------------------------------------------------
public void testTest() {
assertEquals("2002-06-09T00:00:00.000Z", new Instant(TEST_TIME_NOW).toString());
assertEquals("2002-04-05T12:24:00.000Z", new Instant(TEST_TIME1).toString());
assertEquals("2003-05-06T14:28:00.000Z", new Instant(TEST_TIME2).toString());
}
//-----------------------------------------------------------------------
public void testGetMillis() {
Duration test = new Duration(0L);
assertEquals(0, test.getMillis());
test = new Duration(1234567890L);
assertEquals(1234567890L, test.getMillis());
}
public void testEqualsHashCode() {
Duration test1 = new Duration(123L);
Duration test2 = new Duration(123L);
assertEquals(true, test1.equals(test2));
assertEquals(true, test2.equals(test1));
assertEquals(true, test1.equals(test1));
assertEquals(true, test2.equals(test2));
assertEquals(true, test1.hashCode() == test2.hashCode());
assertEquals(true, test1.hashCode() == test1.hashCode());
assertEquals(true, test2.hashCode() == test2.hashCode());
Duration test3 = new Duration(321L);
assertEquals(false, test1.equals(test3));
assertEquals(false, test2.equals(test3));
assertEquals(false, test3.equals(test1));
assertEquals(false, test3.equals(test2));
assertEquals(false, test1.hashCode() == test3.hashCode());
assertEquals(false, test2.hashCode() == test3.hashCode());
assertEquals(false, test1.equals("Hello"));
assertEquals(true, test1.equals(new MockDuration(123L)));
}
class MockDuration extends AbstractDuration {
private final long iValue;
public MockDuration(long value) {
super();
iValue = value;
}
public long getMillis() {
return iValue;
}
}
public void testCompareTo() {
Duration test1 = new Duration(123L);
Duration test1a = new Duration(123L);
assertEquals(0, test1.compareTo(test1a));
assertEquals(0, test1a.compareTo(test1));
assertEquals(0, test1.compareTo(test1));
assertEquals(0, test1a.compareTo(test1a));
Duration test2 = new Duration(321L);
assertEquals(-1, test1.compareTo(test2));
assertEquals(+1, test2.compareTo(test1));
assertEquals(+1, test2.compareTo(new MockDuration(123L)));
assertEquals(0, test1.compareTo(new MockDuration(123L)));
try {
test1.compareTo(null);
fail();
} catch (NullPointerException ex) {}
// try {
// test1.compareTo(new Long(123L));
// fail();
// } catch (ClassCastException ex) {}
}
public void testIsEqual() {
Duration test1 = new Duration(123L);
Duration test1a = new Duration(123L);
assertEquals(true, test1.isEqual(test1a));
assertEquals(true, test1a.isEqual(test1));
assertEquals(true, test1.isEqual(test1));
assertEquals(true, test1a.isEqual(test1a));
Duration test2 = new Duration(321L);
assertEquals(false, test1.isEqual(test2));
assertEquals(false, test2.isEqual(test1));
assertEquals(false, test2.isEqual(new MockDuration(123L)));
assertEquals(true, test1.isEqual(new MockDuration(123L)));
assertEquals(false, test1.isEqual(null));
assertEquals(true, new Duration(0L).isEqual(null));
}
public void testIsBefore() {
Duration test1 = new Duration(123L);
Duration test1a = new Duration(123L);
assertEquals(false, test1.isShorterThan(test1a));
assertEquals(false, test1a.isShorterThan(test1));
assertEquals(false, test1.isShorterThan(test1));
assertEquals(false, test1a.isShorterThan(test1a));
Duration test2 = new Duration(321L);
assertEquals(true, test1.isShorterThan(test2));
assertEquals(false, test2.isShorterThan(test1));
assertEquals(false, test2.isShorterThan(new MockDuration(123L)));
assertEquals(false, test1.isShorterThan(new MockDuration(123L)));
assertEquals(false, test1.isShorterThan(null));
assertEquals(false, new Duration(0L).isShorterThan(null));
}
public void testIsAfter() {
Duration test1 = new Duration(123L);
Duration test1a = new Duration(123L);
assertEquals(false, test1.isLongerThan(test1a));
assertEquals(false, test1a.isLongerThan(test1));
assertEquals(false, test1.isLongerThan(test1));
assertEquals(false, test1a.isLongerThan(test1a));
Duration test2 = new Duration(321L);
assertEquals(false, test1.isLongerThan(test2));
assertEquals(true, test2.isLongerThan(test1));
assertEquals(true, test2.isLongerThan(new MockDuration(123L)));
assertEquals(false, test1.isLongerThan(new MockDuration(123L)));
assertEquals(true, test1.isLongerThan(null));
assertEquals(false, new Duration(0L).isLongerThan(null));
}
//-----------------------------------------------------------------------
public void testSerialization() throws Exception {
Duration test = new Duration(123L);
ByteArrayOutputStream baos = new ByteArrayOutputStream();
ObjectOutputStream oos = new ObjectOutputStream(baos);
oos.writeObject(test);
byte[] bytes = baos.toByteArray();
oos.close();
ByteArrayInputStream bais = new ByteArrayInputStream(bytes);
ObjectInputStream ois = new ObjectInputStream(bais);
Duration result = (Duration) ois.readObject();
ois.close();
assertEquals(test, result);
}
//-----------------------------------------------------------------------
public void testGetStandardSeconds() {
Duration test = new Duration(0L);
assertEquals(0, test.getStandardSeconds());
test = new Duration(1L);
assertEquals(0, test.getStandardSeconds());
test = new Duration(999L);
assertEquals(0, test.getStandardSeconds());
test = new Duration(1000L);
assertEquals(1, test.getStandardSeconds());
test = new Duration(1001L);
assertEquals(1, test.getStandardSeconds());
test = new Duration(1999L);
assertEquals(1, test.getStandardSeconds());
test = new Duration(2000L);
assertEquals(2, test.getStandardSeconds());
test = new Duration(-1L);
assertEquals(0, test.getStandardSeconds());
test = new Duration(-999L);
assertEquals(0, test.getStandardSeconds());
test = new Duration(-1000L);
assertEquals(-1, test.getStandardSeconds());
}
//-----------------------------------------------------------------------
public void testToString() {
long length = (365L + 2L * 30L + 3L * 7L + 4L) * DateTimeConstants.MILLIS_PER_DAY +
5L * DateTimeConstants.MILLIS_PER_HOUR +
6L * DateTimeConstants.MILLIS_PER_MINUTE +
7L * DateTimeConstants.MILLIS_PER_SECOND + 845L;
Duration test = new Duration(length);
assertEquals("PT" + (length / 1000) + "." + (length % 1000) + "S", test.toString());
assertEquals("PT0S", new Duration(0L).toString());
assertEquals("PT10S", new Duration(10000L).toString());
assertEquals("PT1S", new Duration(1000L).toString());
assertEquals("PT12.345S", new Duration(12345L).toString());
assertEquals("PT-12.345S", new Duration(-12345L).toString());
assertEquals("PT-1.123S", new Duration(-1123L).toString());
assertEquals("PT-0.123S", new Duration(-123L).toString());
assertEquals("PT-0.012S", new Duration(-12L).toString());
assertEquals("PT-0.001S", new Duration(-1L).toString());
}
//-----------------------------------------------------------------------
public void testToDuration1() {
Duration test = new Duration(123L);
Duration result = test.toDuration();
assertSame(test, result);
}
public void testToDuration2() {
MockDuration test = new MockDuration(123L);
Duration result = test.toDuration();
assertNotSame(test, result);
assertEquals(test, result);
}
//-----------------------------------------------------------------------
public void testToStandardDays() {
Duration test = new Duration(0L);
assertEquals(Days.days(0), test.toStandardDays());
test = new Duration(1L);
assertEquals(Days.days(0), test.toStandardDays());
test = new Duration(24 * 60 * 60000L - 1);
assertEquals(Days.days(0), test.toStandardDays());
test = new Duration(24 * 60 * 60000L);
assertEquals(Days.days(1), test.toStandardDays());
test = new Duration(24 * 60 * 60000L + 1);
assertEquals(Days.days(1), test.toStandardDays());
test = new Duration(2 * 24 * 60 * 60000L - 1);
assertEquals(Days.days(1), test.toStandardDays());
test = new Duration(2 * 24 * 60 * 60000L);
assertEquals(Days.days(2), test.toStandardDays());
test = new Duration(-1L);
assertEquals(Days.days(0), test.toStandardDays());
test = new Duration(-24 * 60 * 60000L + 1);
assertEquals(Days.days(0), test.toStandardDays());
test = new Duration(-24 * 60 * 60000L);
assertEquals(Days.days(-1), test.toStandardDays());
}
public void testToStandardDays_overflow() {
Duration test = new Duration((((long) Integer.MAX_VALUE) + 1) * 24L * 60L * 60000L);
try {
test.toStandardDays();
fail();
} catch (ArithmeticException ex) {
// expected
}
}
//-----------------------------------------------------------------------
public void testToStandardHours() {
Duration test = new Duration(0L);
assertEquals(Hours.hours(0), test.toStandardHours());
test = new Duration(1L);
assertEquals(Hours.hours(0), test.toStandardHours());
test = new Duration(3600000L - 1);
assertEquals(Hours.hours(0), test.toStandardHours());
test = new Duration(3600000L);
assertEquals(Hours.hours(1), test.toStandardHours());
test = new Duration(3600000L + 1);
assertEquals(Hours.hours(1), test.toStandardHours());
test = new Duration(2 * 3600000L - 1);
assertEquals(Hours.hours(1), test.toStandardHours());
test = new Duration(2 * 3600000L);
assertEquals(Hours.hours(2), test.toStandardHours());
test = new Duration(-1L);
assertEquals(Hours.hours(0), test.toStandardHours());
test = new Duration(-3600000L + 1);
assertEquals(Hours.hours(0), test.toStandardHours());
test = new Duration(-3600000L);
assertEquals(Hours.hours(-1), test.toStandardHours());
}
public void testToStandardHours_overflow() {
Duration test = new Duration(((long) Integer.MAX_VALUE) * 3600000L + 3600000L);
try {
test.toStandardHours();
fail();
} catch (ArithmeticException ex) {
// expected
}
}
//-----------------------------------------------------------------------
public void testToStandardMinutes() {
Duration test = new Duration(0L);
assertEquals(Minutes.minutes(0), test.toStandardMinutes());
test = new Duration(1L);
assertEquals(Minutes.minutes(0), test.toStandardMinutes());
test = new Duration(60000L - 1);
assertEquals(Minutes.minutes(0), test.toStandardMinutes());
test = new Duration(60000L);
assertEquals(Minutes.minutes(1), test.toStandardMinutes());
test = new Duration(60000L + 1);
assertEquals(Minutes.minutes(1), test.toStandardMinutes());
test = new Duration(2 * 60000L - 1);
assertEquals(Minutes.minutes(1), test.toStandardMinutes());
test = new Duration(2 * 60000L);
assertEquals(Minutes.minutes(2), test.toStandardMinutes());
test = new Duration(-1L);
assertEquals(Minutes.minutes(0), test.toStandardMinutes());
test = new Duration(-60000L + 1);
assertEquals(Minutes.minutes(0), test.toStandardMinutes());
test = new Duration(-60000L);
assertEquals(Minutes.minutes(-1), test.toStandardMinutes());
}
public void testToStandardMinutes_overflow() {
Duration test = new Duration(((long) Integer.MAX_VALUE) * 60000L + 60000L);
try {
test.toStandardMinutes();
fail();
} catch (ArithmeticException ex) {
// expected
}
}
//-----------------------------------------------------------------------
public void testToStandardSeconds() {
Duration test = new Duration(0L);
assertEquals(Seconds.seconds(0), test.toStandardSeconds());
test = new Duration(1L);
assertEquals(Seconds.seconds(0), test.toStandardSeconds());
test = new Duration(999L);
assertEquals(Seconds.seconds(0), test.toStandardSeconds());
test = new Duration(1000L);
assertEquals(Seconds.seconds(1), test.toStandardSeconds());
test = new Duration(1001L);
assertEquals(Seconds.seconds(1), test.toStandardSeconds());
test = new Duration(1999L);
assertEquals(Seconds.seconds(1), test.toStandardSeconds());
test = new Duration(2000L);
assertEquals(Seconds.seconds(2), test.toStandardSeconds());
test = new Duration(-1L);
assertEquals(Seconds.seconds(0), test.toStandardSeconds());
test = new Duration(-999L);
assertEquals(Seconds.seconds(0), test.toStandardSeconds());
test = new Duration(-1000L);
assertEquals(Seconds.seconds(-1), test.toStandardSeconds());
}
public void testToStandardSeconds_overflow() {
Duration test = new Duration(((long) Integer.MAX_VALUE) * 1000L + 1000L);
try {
test.toStandardSeconds();
fail();
} catch (ArithmeticException ex) {
// expected
}
}
//-----------------------------------------------------------------------
public void testToPeriod() {
DateTimeZone zone = DateTimeZone.getDefault();
try {
DateTimeZone.setDefault(DateTimeZone.forID("Europe/Paris"));
long length =
(4L + (3L * 7L) + (2L * 30L) + 365L) * DateTimeConstants.MILLIS_PER_DAY +
5L * DateTimeConstants.MILLIS_PER_HOUR +
6L * DateTimeConstants.MILLIS_PER_MINUTE +
7L * DateTimeConstants.MILLIS_PER_SECOND + 8L;
Duration dur = new Duration(length);
Period test = dur.toPeriod();
assertEquals(0, test.getYears()); // (4 + (3 * 7) + (2 * 30) + 365) == 450
assertEquals(0, test.getMonths());
assertEquals(0, test.getWeeks());
assertEquals(0, test.getDays());
assertEquals((450 * 24) + 5, test.getHours());
assertEquals(6, test.getMinutes());
assertEquals(7, test.getSeconds());
assertEquals(8, test.getMillis());
} finally {
DateTimeZone.setDefault(zone);
}
}
public void testToPeriod_fixedZone() throws Throwable {
DateTimeZone zone = DateTimeZone.getDefault();
try {
DateTimeZone.setDefault(DateTimeZone.forOffsetHours(2));
long length =
(4L + (3L * 7L) + (2L * 30L) + 365L) * DateTimeConstants.MILLIS_PER_DAY +
5L * DateTimeConstants.MILLIS_PER_HOUR +
6L * DateTimeConstants.MILLIS_PER_MINUTE +
7L * DateTimeConstants.MILLIS_PER_SECOND + 8L;
Duration dur = new Duration(length);
Period test = dur.toPeriod();
assertEquals(0, test.getYears()); // (4 + (3 * 7) + (2 * 30) + 365) == 450
assertEquals(0, test.getMonths());
assertEquals(0, test.getWeeks());
assertEquals(0, test.getDays());
assertEquals((450 * 24) + 5, test.getHours());
assertEquals(6, test.getMinutes());
assertEquals(7, test.getSeconds());
assertEquals(8, test.getMillis());
} finally {
DateTimeZone.setDefault(zone);
}
}
//-----------------------------------------------------------------------
public void testToPeriod_PeriodType() {
long length =
(4L + (3L * 7L) + (2L * 30L) + 365L) * DateTimeConstants.MILLIS_PER_DAY +
5L * DateTimeConstants.MILLIS_PER_HOUR +
6L * DateTimeConstants.MILLIS_PER_MINUTE +
7L * DateTimeConstants.MILLIS_PER_SECOND + 8L;
Duration test = new Duration(length);
Period result = test.toPeriod(PeriodType.standard().withMillisRemoved());
assertEquals(new Period(test, PeriodType.standard().withMillisRemoved()), result);
assertEquals(new Period(test.getMillis(), PeriodType.standard().withMillisRemoved()), result);
}
//-----------------------------------------------------------------------
public void testToPeriod_Chronology() {
long length =
(4L + (3L * 7L) + (2L * 30L) + 365L) * DateTimeConstants.MILLIS_PER_DAY +
5L * DateTimeConstants.MILLIS_PER_HOUR +
6L * DateTimeConstants.MILLIS_PER_MINUTE +
7L * DateTimeConstants.MILLIS_PER_SECOND + 8L;
Duration test = new Duration(length);
Period result = test.toPeriod(ISOChronology.getInstanceUTC());
assertEquals(new Period(test, ISOChronology.getInstanceUTC()), result);
assertEquals(new Period(test.getMillis(), ISOChronology.getInstanceUTC()), result);
}
//-----------------------------------------------------------------------
public void testToPeriod_PeriodType_Chronology() {
long length =
(4L + (3L * 7L) + (2L * 30L) + 365L) * DateTimeConstants.MILLIS_PER_DAY +
5L * DateTimeConstants.MILLIS_PER_HOUR +
6L * DateTimeConstants.MILLIS_PER_MINUTE +
7L * DateTimeConstants.MILLIS_PER_SECOND + 8L;
Duration test = new Duration(length);
Period result = test.toPeriod(PeriodType.standard().withMillisRemoved(), ISOChronology.getInstanceUTC());
assertEquals(new Period(test, PeriodType.standard().withMillisRemoved(), ISOChronology.getInstanceUTC()), result);
assertEquals(new Period(test.getMillis(), PeriodType.standard().withMillisRemoved(), ISOChronology.getInstanceUTC()), result);
}
//-----------------------------------------------------------------------
public void testToPeriodFrom() {
long length =
(4L + (3L * 7L) + (2L * 30L) + 365L) * DateTimeConstants.MILLIS_PER_DAY +
5L * DateTimeConstants.MILLIS_PER_HOUR +
6L * DateTimeConstants.MILLIS_PER_MINUTE +
7L * DateTimeConstants.MILLIS_PER_SECOND + 8L;
Duration test = new Duration(length);
DateTime dt = new DateTime(2004, 6, 9, 0, 0, 0, 0);
Period result = test.toPeriodFrom(dt);
assertEquals(new Period(dt, test), result);
}
//-----------------------------------------------------------------------
public void testToPeriodFrom_PeriodType() {
long length =
(4L + (3L * 7L) + (2L * 30L) + 365L) * DateTimeConstants.MILLIS_PER_DAY +
5L * DateTimeConstants.MILLIS_PER_HOUR +
6L * DateTimeConstants.MILLIS_PER_MINUTE +
7L * DateTimeConstants.MILLIS_PER_SECOND + 8L;
Duration test = new Duration(length);
DateTime dt = new DateTime(2004, 6, 9, 0, 0, 0, 0);
Period result = test.toPeriodFrom(dt, PeriodType.standard().withMillisRemoved());
assertEquals(new Period(dt, test, PeriodType.standard().withMillisRemoved()), result);
}
//-----------------------------------------------------------------------
public void testToPeriodTo() {
long length =
(4L + (3L * 7L) + (2L * 30L) + 365L) * DateTimeConstants.MILLIS_PER_DAY +
5L * DateTimeConstants.MILLIS_PER_HOUR +
6L * DateTimeConstants.MILLIS_PER_MINUTE +
7L * DateTimeConstants.MILLIS_PER_SECOND + 8L;
Duration test = new Duration(length);
DateTime dt = new DateTime(2004, 6, 9, 0, 0, 0, 0);
Period result = test.toPeriodTo(dt);
assertEquals(new Period(test, dt), result);
}
//-----------------------------------------------------------------------
public void testToPeriodTo_PeriodType() {
long length =
(4L + (3L * 7L) + (2L * 30L) + 365L) * DateTimeConstants.MILLIS_PER_DAY +
5L * DateTimeConstants.MILLIS_PER_HOUR +
6L * DateTimeConstants.MILLIS_PER_MINUTE +
7L * DateTimeConstants.MILLIS_PER_SECOND + 8L;
Duration test = new Duration(length);
DateTime dt = new DateTime(2004, 6, 9, 0, 0, 0, 0);
Period result = test.toPeriodTo(dt, PeriodType.standard().withMillisRemoved());
assertEquals(new Period(test, dt, PeriodType.standard().withMillisRemoved()), result);
}
//-----------------------------------------------------------------------
public void testToIntervalFrom() {
long length =
(4L + (3L * 7L) + (2L * 30L) + 365L) * DateTimeConstants.MILLIS_PER_DAY +
5L * DateTimeConstants.MILLIS_PER_HOUR +
6L * DateTimeConstants.MILLIS_PER_MINUTE +
7L * DateTimeConstants.MILLIS_PER_SECOND + 8L;
Duration test = new Duration(length);
DateTime dt = new DateTime(2004, 6, 9, 0, 0, 0, 0);
Interval result = test.toIntervalFrom(dt);
assertEquals(new Interval(dt, test), result);
}
//-----------------------------------------------------------------------
public void testToIntervalTo() {
long length =
(4L + (3L * 7L) + (2L * 30L) + 365L) * DateTimeConstants.MILLIS_PER_DAY +
5L * DateTimeConstants.MILLIS_PER_HOUR +
6L * DateTimeConstants.MILLIS_PER_MINUTE +
7L * DateTimeConstants.MILLIS_PER_SECOND + 8L;
Duration test = new Duration(length);
DateTime dt = new DateTime(2004, 6, 9, 0, 0, 0, 0);
Interval result = test.toIntervalTo(dt);
assertEquals(new Interval(test, dt), result);
}
//-----------------------------------------------------------------------
public void testWithMillis1() {
Duration test = new Duration(123L);
Duration result = test.withMillis(123L);
assertSame(test, result);
}
public void testWithMillis2() {
Duration test = new Duration(123L);
Duration result = test.withMillis(1234567890L);
assertEquals(1234567890L, result.getMillis());
}
//-----------------------------------------------------------------------
public void testWithDurationAdded_long_int1() {
Duration test = new Duration(123L);
Duration result = test.withDurationAdded(8000L, 1);
assertEquals(8123L, result.getMillis());
}
public void testWithDurationAdded_long_int2() {
Duration test = new Duration(123L);
Duration result = test.withDurationAdded(8000L, 2);
assertEquals(16123L, result.getMillis());
}
public void testWithDurationAdded_long_int3() {
Duration test = new Duration(123L);
Duration result = test.withDurationAdded(8000L, -1);
assertEquals((123L - 8000L), result.getMillis());
}
public void testWithDurationAdded_long_int4() {
Duration test = new Duration(123L);
Duration result = test.withDurationAdded(0L, 1);
assertSame(test, result);
}
public void testWithDurationAdded_long_int5() {
Duration test = new Duration(123L);
Duration result = test.withDurationAdded(8000L, 0);
assertSame(test, result);
}
//-----------------------------------------------------------------------
public void testPlus_long1() {
Duration test = new Duration(123L);
Duration result = test.plus(8000L);
assertEquals(8123L, result.getMillis());
}
public void testPlus_long2() {
Duration test = new Duration(123L);
Duration result = test.plus(0L);
assertSame(test, result);
}
//-----------------------------------------------------------------------
public void testMinus_long1() {
Duration test = new Duration(123L);
Duration result = test.minus(8000L);
assertEquals(123L - 8000L, result.getMillis());
}
public void testMinus_long2() {
Duration test = new Duration(123L);
Duration result = test.minus(0L);
assertSame(test, result);
}
//-----------------------------------------------------------------------
public void testWithDurationAdded_RD_int1() {
Duration test = new Duration(123L);
Duration result = test.withDurationAdded(new Duration(8000L), 1);
assertEquals(8123L, result.getMillis());
}
public void testWithDurationAdded_RD_int2() {
Duration test = new Duration(123L);
Duration result = test.withDurationAdded(new Duration(8000L), 2);
assertEquals(16123L, result.getMillis());
}
public void testWithDurationAdded_RD_int3() {
Duration test = new Duration(123L);
Duration result = test.withDurationAdded(new Duration(8000L), -1);
assertEquals((123L - 8000L), result.getMillis());
}
public void testWithDurationAdded_RD_int4() {
Duration test = new Duration(123L);
Duration result = test.withDurationAdded(new Duration(0L), 1);
assertSame(test, result);
}
public void testWithDurationAdded_RD_int5() {
Duration test = new Duration(123L);
Duration result = test.withDurationAdded(new Duration(8000L), 0);
assertSame(test, result);
}
public void testWithDurationAdded_RD_int6() {
Duration test = new Duration(123L);
Duration result = test.withDurationAdded(null, 0);
assertSame(test, result);
}
//-----------------------------------------------------------------------
public void testPlus_RD1() {
Duration test = new Duration(123L);
Duration result = test.plus(new Duration(8000L));
assertEquals(8123L, result.getMillis());
}
public void testPlus_RD2() {
Duration test = new Duration(123L);
Duration result = test.plus(new Duration(0L));
assertSame(test, result);
}
public void testPlus_RD3() {
Duration test = new Duration(123L);
Duration result = test.plus(null);
assertSame(test, result);
}
//-----------------------------------------------------------------------
public void testMinus_RD1() {
Duration test = new Duration(123L);
Duration result = test.minus(new Duration(8000L));
assertEquals(123L - 8000L, result.getMillis());
}
public void testMinus_RD2() {
Duration test = new Duration(123L);
Duration result = test.minus(new Duration(0L));
assertSame(test, result);
}
public void testMinus_RD3() {
Duration test = new Duration(123L);
Duration result = test.minus(null);
assertSame(test, result);
}
//-----------------------------------------------------------------------
public void testMutableDuration() {
// no MutableDuration, so...
MockMutableDuration test = new MockMutableDuration(123L);
assertEquals(123L, test.getMillis());
test.setMillis(2345L);
assertEquals(2345L, test.getMillis());
}
static class MockMutableDuration extends BaseDuration {
public MockMutableDuration(long duration) {
super(duration);
}
public void setMillis(long duration) {
super.setMillis(duration);
}
}
} | // You are a professional Java test case writer, please create a test case named `testToPeriod_fixedZone` for the issue `Time-113`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Time-113
//
// ## Issue-Title:
// #113 Duration.toPeriod with fixed time zones.
//
//
//
//
//
// ## Issue-Description:
// I have a question concerning the conversion of a Duration to Period. I'm not sure if this is a bug, or if there is a different way to do this.
//
//
// The basis of the problem, is that using Duration.toPeriod() uses the chronology of the default time zone to do the conversion. This can cause different results from a timezone with DST and one without. This can be reproduced easily with this test.
//
//
//
// ```
// //set default time zone with this argument -Duser.timezone="GMT"
// public void testForJodaForum()
// {
// System.out.println("Timezone: " + DateTimeZone.getDefault());
//
// //Duration of more than 24 hours
// Duration aDuration = new Duration(DateTimeConstants.MILLIS\_PER\_HOUR \* 30 + DateTimeConstants.MILLIS\_PER\_MINUTE \* 50
// + DateTimeConstants.MILLIS\_PER\_SECOND \* 14);
//
// System.out.println("Duration before: " + aDuration);
// Period period = aDuration.toPeriod();
// System.out.println("Period after: " + period);
// }
//
// ```
//
// A fixed time zone produces this output
//
// Timezone: Etc/GMT
//
// Duration before: PT111014S
//
// Period after: P1DT6H50M14S
//
//
// A DST time zone produces this output
//
// Timezone: America/Chicago
//
// Duration before: PT111014S
//
// Period after: PT30H50M14S
//
//
// In the joda code, Duration.toPeriod() uses a period constructor that takes the chronology, but null is passed in, so the chronology of the default time zone is used, which leads to this behavior.
//
//
// The javadoc of toPeriod() states that only precise fields of hours, minutes, seconds, and millis will be converted. But for a fixed timezone, days and weeks are also precise, which is stated in the javadoc for toPeriod(Chronology chrono). In our app, we need consistent behavior regardless of the default time zone, which is to have all the extra hours put into the hours bucket. Since Duration is supposed to be a 'time zone independent' length of time, I don't think we should have to do any chronology manipulation to get this to work.
//
//
// Any help is appreciated.
//
//
// Thanks,
//
// Cameron
//
//
//
//
public void testToPeriod_fixedZone() throws Throwable {
| 491 | 22 | 469 | src/test/java/org/joda/time/TestDuration_Basics.java | src/test/java | ```markdown
## Issue-ID: Time-113
## Issue-Title:
#113 Duration.toPeriod with fixed time zones.
## Issue-Description:
I have a question concerning the conversion of a Duration to Period. I'm not sure if this is a bug, or if there is a different way to do this.
The basis of the problem, is that using Duration.toPeriod() uses the chronology of the default time zone to do the conversion. This can cause different results from a timezone with DST and one without. This can be reproduced easily with this test.
```
//set default time zone with this argument -Duser.timezone="GMT"
public void testForJodaForum()
{
System.out.println("Timezone: " + DateTimeZone.getDefault());
//Duration of more than 24 hours
Duration aDuration = new Duration(DateTimeConstants.MILLIS\_PER\_HOUR \* 30 + DateTimeConstants.MILLIS\_PER\_MINUTE \* 50
+ DateTimeConstants.MILLIS\_PER\_SECOND \* 14);
System.out.println("Duration before: " + aDuration);
Period period = aDuration.toPeriod();
System.out.println("Period after: " + period);
}
```
A fixed time zone produces this output
Timezone: Etc/GMT
Duration before: PT111014S
Period after: P1DT6H50M14S
A DST time zone produces this output
Timezone: America/Chicago
Duration before: PT111014S
Period after: PT30H50M14S
In the joda code, Duration.toPeriod() uses a period constructor that takes the chronology, but null is passed in, so the chronology of the default time zone is used, which leads to this behavior.
The javadoc of toPeriod() states that only precise fields of hours, minutes, seconds, and millis will be converted. But for a fixed timezone, days and weeks are also precise, which is stated in the javadoc for toPeriod(Chronology chrono). In our app, we need consistent behavior regardless of the default time zone, which is to have all the extra hours put into the hours bucket. Since Duration is supposed to be a 'time zone independent' length of time, I don't think we should have to do any chronology manipulation to get this to work.
Any help is appreciated.
Thanks,
Cameron
```
You are a professional Java test case writer, please create a test case named `testToPeriod_fixedZone` for the issue `Time-113`, utilizing the provided issue report information and the following function signature.
```java
public void testToPeriod_fixedZone() throws Throwable {
```
| 469 | [
"org.joda.time.base.BasePeriod"
] | 9a00714dd02e407c62904ee6d9da8d2c3ff57ff9c385c175d8d1c054fc21e488 | public void testToPeriod_fixedZone() throws Throwable | // You are a professional Java test case writer, please create a test case named `testToPeriod_fixedZone` for the issue `Time-113`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Time-113
//
// ## Issue-Title:
// #113 Duration.toPeriod with fixed time zones.
//
//
//
//
//
// ## Issue-Description:
// I have a question concerning the conversion of a Duration to Period. I'm not sure if this is a bug, or if there is a different way to do this.
//
//
// The basis of the problem, is that using Duration.toPeriod() uses the chronology of the default time zone to do the conversion. This can cause different results from a timezone with DST and one without. This can be reproduced easily with this test.
//
//
//
// ```
// //set default time zone with this argument -Duser.timezone="GMT"
// public void testForJodaForum()
// {
// System.out.println("Timezone: " + DateTimeZone.getDefault());
//
// //Duration of more than 24 hours
// Duration aDuration = new Duration(DateTimeConstants.MILLIS\_PER\_HOUR \* 30 + DateTimeConstants.MILLIS\_PER\_MINUTE \* 50
// + DateTimeConstants.MILLIS\_PER\_SECOND \* 14);
//
// System.out.println("Duration before: " + aDuration);
// Period period = aDuration.toPeriod();
// System.out.println("Period after: " + period);
// }
//
// ```
//
// A fixed time zone produces this output
//
// Timezone: Etc/GMT
//
// Duration before: PT111014S
//
// Period after: P1DT6H50M14S
//
//
// A DST time zone produces this output
//
// Timezone: America/Chicago
//
// Duration before: PT111014S
//
// Period after: PT30H50M14S
//
//
// In the joda code, Duration.toPeriod() uses a period constructor that takes the chronology, but null is passed in, so the chronology of the default time zone is used, which leads to this behavior.
//
//
// The javadoc of toPeriod() states that only precise fields of hours, minutes, seconds, and millis will be converted. But for a fixed timezone, days and weeks are also precise, which is stated in the javadoc for toPeriod(Chronology chrono). In our app, we need consistent behavior regardless of the default time zone, which is to have all the extra hours put into the hours bucket. Since Duration is supposed to be a 'time zone independent' length of time, I don't think we should have to do any chronology manipulation to get this to work.
//
//
// Any help is appreciated.
//
//
// Thanks,
//
// Cameron
//
//
//
//
| Time | /*
* Copyright 2001-2009 Stephen Colebourne
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.joda.time;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.util.Locale;
import java.util.TimeZone;
import junit.framework.TestCase;
import junit.framework.TestSuite;
import org.joda.time.base.AbstractDuration;
import org.joda.time.base.BaseDuration;
import org.joda.time.chrono.ISOChronology;
/**
* This class is a Junit unit test for Duration.
*
* @author Stephen Colebourne
*/
public class TestDuration_Basics extends TestCase {
// Test in 2002/03 as time zones are more well known
// (before the late 90's they were all over the place)
private static final DateTimeZone LONDON = DateTimeZone.forID("Europe/London");
long y2002days = 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 +
365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365;
long y2003days = 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 +
365 + 365 + 366 + 365 + 365 + 365 + 366 + 365 + 365 + 365 +
366 + 365 + 365;
// 2002-06-09
private long TEST_TIME_NOW =
(y2002days + 31L + 28L + 31L + 30L + 31L + 9L -1L) * DateTimeConstants.MILLIS_PER_DAY;
// 2002-04-05
private long TEST_TIME1 =
(y2002days + 31L + 28L + 31L + 5L -1L) * DateTimeConstants.MILLIS_PER_DAY
+ 12L * DateTimeConstants.MILLIS_PER_HOUR
+ 24L * DateTimeConstants.MILLIS_PER_MINUTE;
// 2003-05-06
private long TEST_TIME2 =
(y2003days + 31L + 28L + 31L + 30L + 6L -1L) * DateTimeConstants.MILLIS_PER_DAY
+ 14L * DateTimeConstants.MILLIS_PER_HOUR
+ 28L * DateTimeConstants.MILLIS_PER_MINUTE;
private DateTimeZone originalDateTimeZone = null;
private TimeZone originalTimeZone = null;
private Locale originalLocale = null;
public static void main(String[] args) {
junit.textui.TestRunner.run(suite());
}
public static TestSuite suite() {
return new TestSuite(TestDuration_Basics.class);
}
public TestDuration_Basics(String name) {
super(name);
}
protected void setUp() throws Exception {
DateTimeUtils.setCurrentMillisFixed(TEST_TIME_NOW);
originalDateTimeZone = DateTimeZone.getDefault();
originalTimeZone = TimeZone.getDefault();
originalLocale = Locale.getDefault();
DateTimeZone.setDefault(LONDON);
TimeZone.setDefault(TimeZone.getTimeZone("Europe/London"));
Locale.setDefault(Locale.UK);
}
protected void tearDown() throws Exception {
DateTimeUtils.setCurrentMillisSystem();
DateTimeZone.setDefault(originalDateTimeZone);
TimeZone.setDefault(originalTimeZone);
Locale.setDefault(originalLocale);
originalDateTimeZone = null;
originalTimeZone = null;
originalLocale = null;
}
//-----------------------------------------------------------------------
public void testTest() {
assertEquals("2002-06-09T00:00:00.000Z", new Instant(TEST_TIME_NOW).toString());
assertEquals("2002-04-05T12:24:00.000Z", new Instant(TEST_TIME1).toString());
assertEquals("2003-05-06T14:28:00.000Z", new Instant(TEST_TIME2).toString());
}
//-----------------------------------------------------------------------
public void testGetMillis() {
Duration test = new Duration(0L);
assertEquals(0, test.getMillis());
test = new Duration(1234567890L);
assertEquals(1234567890L, test.getMillis());
}
public void testEqualsHashCode() {
Duration test1 = new Duration(123L);
Duration test2 = new Duration(123L);
assertEquals(true, test1.equals(test2));
assertEquals(true, test2.equals(test1));
assertEquals(true, test1.equals(test1));
assertEquals(true, test2.equals(test2));
assertEquals(true, test1.hashCode() == test2.hashCode());
assertEquals(true, test1.hashCode() == test1.hashCode());
assertEquals(true, test2.hashCode() == test2.hashCode());
Duration test3 = new Duration(321L);
assertEquals(false, test1.equals(test3));
assertEquals(false, test2.equals(test3));
assertEquals(false, test3.equals(test1));
assertEquals(false, test3.equals(test2));
assertEquals(false, test1.hashCode() == test3.hashCode());
assertEquals(false, test2.hashCode() == test3.hashCode());
assertEquals(false, test1.equals("Hello"));
assertEquals(true, test1.equals(new MockDuration(123L)));
}
class MockDuration extends AbstractDuration {
private final long iValue;
public MockDuration(long value) {
super();
iValue = value;
}
public long getMillis() {
return iValue;
}
}
public void testCompareTo() {
Duration test1 = new Duration(123L);
Duration test1a = new Duration(123L);
assertEquals(0, test1.compareTo(test1a));
assertEquals(0, test1a.compareTo(test1));
assertEquals(0, test1.compareTo(test1));
assertEquals(0, test1a.compareTo(test1a));
Duration test2 = new Duration(321L);
assertEquals(-1, test1.compareTo(test2));
assertEquals(+1, test2.compareTo(test1));
assertEquals(+1, test2.compareTo(new MockDuration(123L)));
assertEquals(0, test1.compareTo(new MockDuration(123L)));
try {
test1.compareTo(null);
fail();
} catch (NullPointerException ex) {}
// try {
// test1.compareTo(new Long(123L));
// fail();
// } catch (ClassCastException ex) {}
}
public void testIsEqual() {
Duration test1 = new Duration(123L);
Duration test1a = new Duration(123L);
assertEquals(true, test1.isEqual(test1a));
assertEquals(true, test1a.isEqual(test1));
assertEquals(true, test1.isEqual(test1));
assertEquals(true, test1a.isEqual(test1a));
Duration test2 = new Duration(321L);
assertEquals(false, test1.isEqual(test2));
assertEquals(false, test2.isEqual(test1));
assertEquals(false, test2.isEqual(new MockDuration(123L)));
assertEquals(true, test1.isEqual(new MockDuration(123L)));
assertEquals(false, test1.isEqual(null));
assertEquals(true, new Duration(0L).isEqual(null));
}
public void testIsBefore() {
Duration test1 = new Duration(123L);
Duration test1a = new Duration(123L);
assertEquals(false, test1.isShorterThan(test1a));
assertEquals(false, test1a.isShorterThan(test1));
assertEquals(false, test1.isShorterThan(test1));
assertEquals(false, test1a.isShorterThan(test1a));
Duration test2 = new Duration(321L);
assertEquals(true, test1.isShorterThan(test2));
assertEquals(false, test2.isShorterThan(test1));
assertEquals(false, test2.isShorterThan(new MockDuration(123L)));
assertEquals(false, test1.isShorterThan(new MockDuration(123L)));
assertEquals(false, test1.isShorterThan(null));
assertEquals(false, new Duration(0L).isShorterThan(null));
}
public void testIsAfter() {
Duration test1 = new Duration(123L);
Duration test1a = new Duration(123L);
assertEquals(false, test1.isLongerThan(test1a));
assertEquals(false, test1a.isLongerThan(test1));
assertEquals(false, test1.isLongerThan(test1));
assertEquals(false, test1a.isLongerThan(test1a));
Duration test2 = new Duration(321L);
assertEquals(false, test1.isLongerThan(test2));
assertEquals(true, test2.isLongerThan(test1));
assertEquals(true, test2.isLongerThan(new MockDuration(123L)));
assertEquals(false, test1.isLongerThan(new MockDuration(123L)));
assertEquals(true, test1.isLongerThan(null));
assertEquals(false, new Duration(0L).isLongerThan(null));
}
//-----------------------------------------------------------------------
public void testSerialization() throws Exception {
Duration test = new Duration(123L);
ByteArrayOutputStream baos = new ByteArrayOutputStream();
ObjectOutputStream oos = new ObjectOutputStream(baos);
oos.writeObject(test);
byte[] bytes = baos.toByteArray();
oos.close();
ByteArrayInputStream bais = new ByteArrayInputStream(bytes);
ObjectInputStream ois = new ObjectInputStream(bais);
Duration result = (Duration) ois.readObject();
ois.close();
assertEquals(test, result);
}
//-----------------------------------------------------------------------
public void testGetStandardSeconds() {
Duration test = new Duration(0L);
assertEquals(0, test.getStandardSeconds());
test = new Duration(1L);
assertEquals(0, test.getStandardSeconds());
test = new Duration(999L);
assertEquals(0, test.getStandardSeconds());
test = new Duration(1000L);
assertEquals(1, test.getStandardSeconds());
test = new Duration(1001L);
assertEquals(1, test.getStandardSeconds());
test = new Duration(1999L);
assertEquals(1, test.getStandardSeconds());
test = new Duration(2000L);
assertEquals(2, test.getStandardSeconds());
test = new Duration(-1L);
assertEquals(0, test.getStandardSeconds());
test = new Duration(-999L);
assertEquals(0, test.getStandardSeconds());
test = new Duration(-1000L);
assertEquals(-1, test.getStandardSeconds());
}
//-----------------------------------------------------------------------
public void testToString() {
long length = (365L + 2L * 30L + 3L * 7L + 4L) * DateTimeConstants.MILLIS_PER_DAY +
5L * DateTimeConstants.MILLIS_PER_HOUR +
6L * DateTimeConstants.MILLIS_PER_MINUTE +
7L * DateTimeConstants.MILLIS_PER_SECOND + 845L;
Duration test = new Duration(length);
assertEquals("PT" + (length / 1000) + "." + (length % 1000) + "S", test.toString());
assertEquals("PT0S", new Duration(0L).toString());
assertEquals("PT10S", new Duration(10000L).toString());
assertEquals("PT1S", new Duration(1000L).toString());
assertEquals("PT12.345S", new Duration(12345L).toString());
assertEquals("PT-12.345S", new Duration(-12345L).toString());
assertEquals("PT-1.123S", new Duration(-1123L).toString());
assertEquals("PT-0.123S", new Duration(-123L).toString());
assertEquals("PT-0.012S", new Duration(-12L).toString());
assertEquals("PT-0.001S", new Duration(-1L).toString());
}
//-----------------------------------------------------------------------
public void testToDuration1() {
Duration test = new Duration(123L);
Duration result = test.toDuration();
assertSame(test, result);
}
public void testToDuration2() {
MockDuration test = new MockDuration(123L);
Duration result = test.toDuration();
assertNotSame(test, result);
assertEquals(test, result);
}
//-----------------------------------------------------------------------
public void testToStandardDays() {
Duration test = new Duration(0L);
assertEquals(Days.days(0), test.toStandardDays());
test = new Duration(1L);
assertEquals(Days.days(0), test.toStandardDays());
test = new Duration(24 * 60 * 60000L - 1);
assertEquals(Days.days(0), test.toStandardDays());
test = new Duration(24 * 60 * 60000L);
assertEquals(Days.days(1), test.toStandardDays());
test = new Duration(24 * 60 * 60000L + 1);
assertEquals(Days.days(1), test.toStandardDays());
test = new Duration(2 * 24 * 60 * 60000L - 1);
assertEquals(Days.days(1), test.toStandardDays());
test = new Duration(2 * 24 * 60 * 60000L);
assertEquals(Days.days(2), test.toStandardDays());
test = new Duration(-1L);
assertEquals(Days.days(0), test.toStandardDays());
test = new Duration(-24 * 60 * 60000L + 1);
assertEquals(Days.days(0), test.toStandardDays());
test = new Duration(-24 * 60 * 60000L);
assertEquals(Days.days(-1), test.toStandardDays());
}
public void testToStandardDays_overflow() {
Duration test = new Duration((((long) Integer.MAX_VALUE) + 1) * 24L * 60L * 60000L);
try {
test.toStandardDays();
fail();
} catch (ArithmeticException ex) {
// expected
}
}
//-----------------------------------------------------------------------
public void testToStandardHours() {
Duration test = new Duration(0L);
assertEquals(Hours.hours(0), test.toStandardHours());
test = new Duration(1L);
assertEquals(Hours.hours(0), test.toStandardHours());
test = new Duration(3600000L - 1);
assertEquals(Hours.hours(0), test.toStandardHours());
test = new Duration(3600000L);
assertEquals(Hours.hours(1), test.toStandardHours());
test = new Duration(3600000L + 1);
assertEquals(Hours.hours(1), test.toStandardHours());
test = new Duration(2 * 3600000L - 1);
assertEquals(Hours.hours(1), test.toStandardHours());
test = new Duration(2 * 3600000L);
assertEquals(Hours.hours(2), test.toStandardHours());
test = new Duration(-1L);
assertEquals(Hours.hours(0), test.toStandardHours());
test = new Duration(-3600000L + 1);
assertEquals(Hours.hours(0), test.toStandardHours());
test = new Duration(-3600000L);
assertEquals(Hours.hours(-1), test.toStandardHours());
}
public void testToStandardHours_overflow() {
Duration test = new Duration(((long) Integer.MAX_VALUE) * 3600000L + 3600000L);
try {
test.toStandardHours();
fail();
} catch (ArithmeticException ex) {
// expected
}
}
//-----------------------------------------------------------------------
public void testToStandardMinutes() {
Duration test = new Duration(0L);
assertEquals(Minutes.minutes(0), test.toStandardMinutes());
test = new Duration(1L);
assertEquals(Minutes.minutes(0), test.toStandardMinutes());
test = new Duration(60000L - 1);
assertEquals(Minutes.minutes(0), test.toStandardMinutes());
test = new Duration(60000L);
assertEquals(Minutes.minutes(1), test.toStandardMinutes());
test = new Duration(60000L + 1);
assertEquals(Minutes.minutes(1), test.toStandardMinutes());
test = new Duration(2 * 60000L - 1);
assertEquals(Minutes.minutes(1), test.toStandardMinutes());
test = new Duration(2 * 60000L);
assertEquals(Minutes.minutes(2), test.toStandardMinutes());
test = new Duration(-1L);
assertEquals(Minutes.minutes(0), test.toStandardMinutes());
test = new Duration(-60000L + 1);
assertEquals(Minutes.minutes(0), test.toStandardMinutes());
test = new Duration(-60000L);
assertEquals(Minutes.minutes(-1), test.toStandardMinutes());
}
public void testToStandardMinutes_overflow() {
Duration test = new Duration(((long) Integer.MAX_VALUE) * 60000L + 60000L);
try {
test.toStandardMinutes();
fail();
} catch (ArithmeticException ex) {
// expected
}
}
//-----------------------------------------------------------------------
public void testToStandardSeconds() {
Duration test = new Duration(0L);
assertEquals(Seconds.seconds(0), test.toStandardSeconds());
test = new Duration(1L);
assertEquals(Seconds.seconds(0), test.toStandardSeconds());
test = new Duration(999L);
assertEquals(Seconds.seconds(0), test.toStandardSeconds());
test = new Duration(1000L);
assertEquals(Seconds.seconds(1), test.toStandardSeconds());
test = new Duration(1001L);
assertEquals(Seconds.seconds(1), test.toStandardSeconds());
test = new Duration(1999L);
assertEquals(Seconds.seconds(1), test.toStandardSeconds());
test = new Duration(2000L);
assertEquals(Seconds.seconds(2), test.toStandardSeconds());
test = new Duration(-1L);
assertEquals(Seconds.seconds(0), test.toStandardSeconds());
test = new Duration(-999L);
assertEquals(Seconds.seconds(0), test.toStandardSeconds());
test = new Duration(-1000L);
assertEquals(Seconds.seconds(-1), test.toStandardSeconds());
}
public void testToStandardSeconds_overflow() {
Duration test = new Duration(((long) Integer.MAX_VALUE) * 1000L + 1000L);
try {
test.toStandardSeconds();
fail();
} catch (ArithmeticException ex) {
// expected
}
}
//-----------------------------------------------------------------------
public void testToPeriod() {
DateTimeZone zone = DateTimeZone.getDefault();
try {
DateTimeZone.setDefault(DateTimeZone.forID("Europe/Paris"));
long length =
(4L + (3L * 7L) + (2L * 30L) + 365L) * DateTimeConstants.MILLIS_PER_DAY +
5L * DateTimeConstants.MILLIS_PER_HOUR +
6L * DateTimeConstants.MILLIS_PER_MINUTE +
7L * DateTimeConstants.MILLIS_PER_SECOND + 8L;
Duration dur = new Duration(length);
Period test = dur.toPeriod();
assertEquals(0, test.getYears()); // (4 + (3 * 7) + (2 * 30) + 365) == 450
assertEquals(0, test.getMonths());
assertEquals(0, test.getWeeks());
assertEquals(0, test.getDays());
assertEquals((450 * 24) + 5, test.getHours());
assertEquals(6, test.getMinutes());
assertEquals(7, test.getSeconds());
assertEquals(8, test.getMillis());
} finally {
DateTimeZone.setDefault(zone);
}
}
public void testToPeriod_fixedZone() throws Throwable {
DateTimeZone zone = DateTimeZone.getDefault();
try {
DateTimeZone.setDefault(DateTimeZone.forOffsetHours(2));
long length =
(4L + (3L * 7L) + (2L * 30L) + 365L) * DateTimeConstants.MILLIS_PER_DAY +
5L * DateTimeConstants.MILLIS_PER_HOUR +
6L * DateTimeConstants.MILLIS_PER_MINUTE +
7L * DateTimeConstants.MILLIS_PER_SECOND + 8L;
Duration dur = new Duration(length);
Period test = dur.toPeriod();
assertEquals(0, test.getYears()); // (4 + (3 * 7) + (2 * 30) + 365) == 450
assertEquals(0, test.getMonths());
assertEquals(0, test.getWeeks());
assertEquals(0, test.getDays());
assertEquals((450 * 24) + 5, test.getHours());
assertEquals(6, test.getMinutes());
assertEquals(7, test.getSeconds());
assertEquals(8, test.getMillis());
} finally {
DateTimeZone.setDefault(zone);
}
}
//-----------------------------------------------------------------------
public void testToPeriod_PeriodType() {
long length =
(4L + (3L * 7L) + (2L * 30L) + 365L) * DateTimeConstants.MILLIS_PER_DAY +
5L * DateTimeConstants.MILLIS_PER_HOUR +
6L * DateTimeConstants.MILLIS_PER_MINUTE +
7L * DateTimeConstants.MILLIS_PER_SECOND + 8L;
Duration test = new Duration(length);
Period result = test.toPeriod(PeriodType.standard().withMillisRemoved());
assertEquals(new Period(test, PeriodType.standard().withMillisRemoved()), result);
assertEquals(new Period(test.getMillis(), PeriodType.standard().withMillisRemoved()), result);
}
//-----------------------------------------------------------------------
public void testToPeriod_Chronology() {
long length =
(4L + (3L * 7L) + (2L * 30L) + 365L) * DateTimeConstants.MILLIS_PER_DAY +
5L * DateTimeConstants.MILLIS_PER_HOUR +
6L * DateTimeConstants.MILLIS_PER_MINUTE +
7L * DateTimeConstants.MILLIS_PER_SECOND + 8L;
Duration test = new Duration(length);
Period result = test.toPeriod(ISOChronology.getInstanceUTC());
assertEquals(new Period(test, ISOChronology.getInstanceUTC()), result);
assertEquals(new Period(test.getMillis(), ISOChronology.getInstanceUTC()), result);
}
//-----------------------------------------------------------------------
public void testToPeriod_PeriodType_Chronology() {
long length =
(4L + (3L * 7L) + (2L * 30L) + 365L) * DateTimeConstants.MILLIS_PER_DAY +
5L * DateTimeConstants.MILLIS_PER_HOUR +
6L * DateTimeConstants.MILLIS_PER_MINUTE +
7L * DateTimeConstants.MILLIS_PER_SECOND + 8L;
Duration test = new Duration(length);
Period result = test.toPeriod(PeriodType.standard().withMillisRemoved(), ISOChronology.getInstanceUTC());
assertEquals(new Period(test, PeriodType.standard().withMillisRemoved(), ISOChronology.getInstanceUTC()), result);
assertEquals(new Period(test.getMillis(), PeriodType.standard().withMillisRemoved(), ISOChronology.getInstanceUTC()), result);
}
//-----------------------------------------------------------------------
public void testToPeriodFrom() {
long length =
(4L + (3L * 7L) + (2L * 30L) + 365L) * DateTimeConstants.MILLIS_PER_DAY +
5L * DateTimeConstants.MILLIS_PER_HOUR +
6L * DateTimeConstants.MILLIS_PER_MINUTE +
7L * DateTimeConstants.MILLIS_PER_SECOND + 8L;
Duration test = new Duration(length);
DateTime dt = new DateTime(2004, 6, 9, 0, 0, 0, 0);
Period result = test.toPeriodFrom(dt);
assertEquals(new Period(dt, test), result);
}
//-----------------------------------------------------------------------
public void testToPeriodFrom_PeriodType() {
long length =
(4L + (3L * 7L) + (2L * 30L) + 365L) * DateTimeConstants.MILLIS_PER_DAY +
5L * DateTimeConstants.MILLIS_PER_HOUR +
6L * DateTimeConstants.MILLIS_PER_MINUTE +
7L * DateTimeConstants.MILLIS_PER_SECOND + 8L;
Duration test = new Duration(length);
DateTime dt = new DateTime(2004, 6, 9, 0, 0, 0, 0);
Period result = test.toPeriodFrom(dt, PeriodType.standard().withMillisRemoved());
assertEquals(new Period(dt, test, PeriodType.standard().withMillisRemoved()), result);
}
//-----------------------------------------------------------------------
public void testToPeriodTo() {
long length =
(4L + (3L * 7L) + (2L * 30L) + 365L) * DateTimeConstants.MILLIS_PER_DAY +
5L * DateTimeConstants.MILLIS_PER_HOUR +
6L * DateTimeConstants.MILLIS_PER_MINUTE +
7L * DateTimeConstants.MILLIS_PER_SECOND + 8L;
Duration test = new Duration(length);
DateTime dt = new DateTime(2004, 6, 9, 0, 0, 0, 0);
Period result = test.toPeriodTo(dt);
assertEquals(new Period(test, dt), result);
}
//-----------------------------------------------------------------------
public void testToPeriodTo_PeriodType() {
long length =
(4L + (3L * 7L) + (2L * 30L) + 365L) * DateTimeConstants.MILLIS_PER_DAY +
5L * DateTimeConstants.MILLIS_PER_HOUR +
6L * DateTimeConstants.MILLIS_PER_MINUTE +
7L * DateTimeConstants.MILLIS_PER_SECOND + 8L;
Duration test = new Duration(length);
DateTime dt = new DateTime(2004, 6, 9, 0, 0, 0, 0);
Period result = test.toPeriodTo(dt, PeriodType.standard().withMillisRemoved());
assertEquals(new Period(test, dt, PeriodType.standard().withMillisRemoved()), result);
}
//-----------------------------------------------------------------------
public void testToIntervalFrom() {
long length =
(4L + (3L * 7L) + (2L * 30L) + 365L) * DateTimeConstants.MILLIS_PER_DAY +
5L * DateTimeConstants.MILLIS_PER_HOUR +
6L * DateTimeConstants.MILLIS_PER_MINUTE +
7L * DateTimeConstants.MILLIS_PER_SECOND + 8L;
Duration test = new Duration(length);
DateTime dt = new DateTime(2004, 6, 9, 0, 0, 0, 0);
Interval result = test.toIntervalFrom(dt);
assertEquals(new Interval(dt, test), result);
}
//-----------------------------------------------------------------------
public void testToIntervalTo() {
long length =
(4L + (3L * 7L) + (2L * 30L) + 365L) * DateTimeConstants.MILLIS_PER_DAY +
5L * DateTimeConstants.MILLIS_PER_HOUR +
6L * DateTimeConstants.MILLIS_PER_MINUTE +
7L * DateTimeConstants.MILLIS_PER_SECOND + 8L;
Duration test = new Duration(length);
DateTime dt = new DateTime(2004, 6, 9, 0, 0, 0, 0);
Interval result = test.toIntervalTo(dt);
assertEquals(new Interval(test, dt), result);
}
//-----------------------------------------------------------------------
public void testWithMillis1() {
Duration test = new Duration(123L);
Duration result = test.withMillis(123L);
assertSame(test, result);
}
public void testWithMillis2() {
Duration test = new Duration(123L);
Duration result = test.withMillis(1234567890L);
assertEquals(1234567890L, result.getMillis());
}
//-----------------------------------------------------------------------
public void testWithDurationAdded_long_int1() {
Duration test = new Duration(123L);
Duration result = test.withDurationAdded(8000L, 1);
assertEquals(8123L, result.getMillis());
}
public void testWithDurationAdded_long_int2() {
Duration test = new Duration(123L);
Duration result = test.withDurationAdded(8000L, 2);
assertEquals(16123L, result.getMillis());
}
public void testWithDurationAdded_long_int3() {
Duration test = new Duration(123L);
Duration result = test.withDurationAdded(8000L, -1);
assertEquals((123L - 8000L), result.getMillis());
}
public void testWithDurationAdded_long_int4() {
Duration test = new Duration(123L);
Duration result = test.withDurationAdded(0L, 1);
assertSame(test, result);
}
public void testWithDurationAdded_long_int5() {
Duration test = new Duration(123L);
Duration result = test.withDurationAdded(8000L, 0);
assertSame(test, result);
}
//-----------------------------------------------------------------------
public void testPlus_long1() {
Duration test = new Duration(123L);
Duration result = test.plus(8000L);
assertEquals(8123L, result.getMillis());
}
public void testPlus_long2() {
Duration test = new Duration(123L);
Duration result = test.plus(0L);
assertSame(test, result);
}
//-----------------------------------------------------------------------
public void testMinus_long1() {
Duration test = new Duration(123L);
Duration result = test.minus(8000L);
assertEquals(123L - 8000L, result.getMillis());
}
public void testMinus_long2() {
Duration test = new Duration(123L);
Duration result = test.minus(0L);
assertSame(test, result);
}
//-----------------------------------------------------------------------
public void testWithDurationAdded_RD_int1() {
Duration test = new Duration(123L);
Duration result = test.withDurationAdded(new Duration(8000L), 1);
assertEquals(8123L, result.getMillis());
}
public void testWithDurationAdded_RD_int2() {
Duration test = new Duration(123L);
Duration result = test.withDurationAdded(new Duration(8000L), 2);
assertEquals(16123L, result.getMillis());
}
public void testWithDurationAdded_RD_int3() {
Duration test = new Duration(123L);
Duration result = test.withDurationAdded(new Duration(8000L), -1);
assertEquals((123L - 8000L), result.getMillis());
}
public void testWithDurationAdded_RD_int4() {
Duration test = new Duration(123L);
Duration result = test.withDurationAdded(new Duration(0L), 1);
assertSame(test, result);
}
public void testWithDurationAdded_RD_int5() {
Duration test = new Duration(123L);
Duration result = test.withDurationAdded(new Duration(8000L), 0);
assertSame(test, result);
}
public void testWithDurationAdded_RD_int6() {
Duration test = new Duration(123L);
Duration result = test.withDurationAdded(null, 0);
assertSame(test, result);
}
//-----------------------------------------------------------------------
public void testPlus_RD1() {
Duration test = new Duration(123L);
Duration result = test.plus(new Duration(8000L));
assertEquals(8123L, result.getMillis());
}
public void testPlus_RD2() {
Duration test = new Duration(123L);
Duration result = test.plus(new Duration(0L));
assertSame(test, result);
}
public void testPlus_RD3() {
Duration test = new Duration(123L);
Duration result = test.plus(null);
assertSame(test, result);
}
//-----------------------------------------------------------------------
public void testMinus_RD1() {
Duration test = new Duration(123L);
Duration result = test.minus(new Duration(8000L));
assertEquals(123L - 8000L, result.getMillis());
}
public void testMinus_RD2() {
Duration test = new Duration(123L);
Duration result = test.minus(new Duration(0L));
assertSame(test, result);
}
public void testMinus_RD3() {
Duration test = new Duration(123L);
Duration result = test.minus(null);
assertSame(test, result);
}
//-----------------------------------------------------------------------
public void testMutableDuration() {
// no MutableDuration, so...
MockMutableDuration test = new MockMutableDuration(123L);
assertEquals(123L, test.getMillis());
test.setMillis(2345L);
assertEquals(2345L, test.getMillis());
}
static class MockMutableDuration extends BaseDuration {
public MockMutableDuration(long duration) {
super(duration);
}
public void setMillis(long duration) {
super.setMillis(duration);
}
}
} |
||
public void testAssignWithCall() {
test("var fun, x; (fun = function(){ x; })();",
"var x; (function(){ x; })();");
} | com.google.javascript.jscomp.NameAnalyzerTest::testAssignWithCall | test/com/google/javascript/jscomp/NameAnalyzerTest.java | 1,237 | test/com/google/javascript/jscomp/NameAnalyzerTest.java | testAssignWithCall | /*
* Copyright 2006 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import com.google.javascript.rhino.Node;
/**
* Tests for {@link NameAnalyzer}
*
*/
public class NameAnalyzerTest extends CompilerTestCase {
private static String kExterns =
"var window, top;" +
"var document;" +
"var Function;" +
"var Array;" +
"var externfoo; methods.externfoo;";
public NameAnalyzerTest() {
super(kExterns);
}
@Override
protected void setUp() {
super.enableNormalize();
super.enableLineNumberCheck(true);
}
@Override
protected int getNumRepetitions() {
// pass reaches steady state after 1 iteration.
return 1;
}
public void testRemoveVarDeclaration1() {
test("var foo = 3;", "");
}
public void testRemoveVarDeclaration2() {
test("var foo = 3, bar = 4; externfoo = foo;",
"var foo = 3; externfoo = foo;");
}
public void testRemoveVarDeclaration3() {
test("var a = f(), b = 1, c = 2; b; c", "f();var b = 1, c = 2; b; c");
}
public void testRemoveVarDeclaration4() {
test("var a = 0, b = f(), c = 2; a; c", "var a = 0;f();var c = 2; a; c");
}
public void testRemoveVarDeclaration5() {
test("var a = 0, b = 1, c = f(); a; b", "var a = 0, b = 1; f(); a; b");
}
public void testRemoveVarDeclaration6() {
test("var a = 0, b = a = 1; a", "var a = 0; a = 1; a");
}
public void testRemoveVarDeclaration7() {
test("var a = 0, b = a = 1", "");
}
public void testRemoveVarDeclaration8() {
test("var a;var b = 0, c = a = b = 1", "");
}
public void testRemoveDeclaration1() {
test("var a;var b = 0, c = a = b = 1", "");
}
public void testRemoveDeclaration2() {
test("var a,b,c; c = a = b = 1", "");
}
public void testRemoveDeclaration3() {
test("var a,b,c; c = a = b = {}; a.x = 1;", "");
}
public void testRemoveDeclaration4() {
testSame("var a,b,c; c = a = b = {}; a.x = 1;alert(c.x);");
}
public void testRemoveDeclaration5() {
test("var a,b,c; c = a = b = null; use(b)", "var b;b=null;use(b)");
}
public void testRemoveDeclaration6() {
test("var a,b,c; c = a = b = 'str';use(b)", "var b;b='str';use(b)");
}
public void testRemoveDeclaration7() {
test("var a,b,c; c = a = b = true;use(b)", "var b;b=true;use(b)");
}
public void testRemoveFunction1() {
test("var foo = function(){};", "");
}
public void testRemoveFunction2() {
test("var foo; foo = function(){};", "");
}
public void testRemoveFunction3() {
test("var foo = {}; foo.bar = function() {};", "");
}
public void testRemoveFunction4() {
test("var a = {}; a.b = {}; a.b.c = function() {};", "");
}
public void testReferredToByWindow() {
testSame("var foo = {}; foo.bar = function() {}; window['fooz'] = foo.bar");
}
public void testExtern() {
testSame("externfoo = 5");
}
public void testRemoveNamedFunction() {
test("function foo(){}", "");
}
public void testRemoveRecursiveFunction1() {
test("function f(){f()}", "");
}
public void testRemoveRecursiveFunction2() {
test("var f = function (){f()}", "");
}
public void testRemoveRecursiveFunction2a() {
test("var f = function g(){g()}", "");
}
public void testRemoveRecursiveFunction3() {
test("var f;f = function (){f()}", "");
}
public void testRemoveRecursiveFunction4() {
// don't removed if name definition doesn't exist.
testSame("f = function (){f()}");
}
public void testRemoveRecursiveFunction5() {
test("function g(){f()}function f(){g()}", "");
}
public void testRemoveRecursiveFunction6() {
test("var f=function(){g()};function g(){f()}", "");
}
public void testRemoveRecursiveFunction7() {
test("var g = function(){f()};var f = function(){g()}", "");
}
public void testRemoveRecursiveFunction8() {
test("var o = {};o.f = function(){o.f()}", "");
}
public void testRemoveRecursiveFunction9() {
testSame("var o = {};o.f = function(){o.f()};o.f()");
}
public void testSideEffectClassification1() {
test("foo();", "foo();");
}
public void testSideEffectClassification2() {
test("var a = foo();", "foo();");
}
public void testSideEffectClassification3() {
testSame("var a = foo();window['b']=a;");
}
public void testSideEffectClassification4() {
testSame("function sef(){} sef();");
}
public void testSideEffectClassification5() {
testSame("function nsef(){} var a = nsef();window['b']=a;");
}
public void testSideEffectClassification6() {
test("function sef(){} sef();", "function sef(){} sef();");
}
public void testSideEffectClassification7() {
testSame("function sef(){} var a = sef();window['b']=a;");
}
public void testNoSideEffectAnnotation1() {
test("function f(){} var a = f();",
"function f(){} f()");
}
public void testNoSideEffectAnnotation2() {
test("/**@nosideeffects*/function f(){}", "var a = f();",
"", null, null);
}
public void testNoSideEffectAnnotation3() {
test("var f = function(){}; var a = f();",
"var f = function(){}; f();");
}
public void testNoSideEffectAnnotation4() {
test("var f = /**@nosideeffects*/function(){};", "var a = f();",
"", null, null);
}
public void testNoSideEffectAnnotation5() {
test("var f; f = function(){}; var a = f();",
"var f; f = function(){}; f();");
}
public void testNoSideEffectAnnotation6() {
test("var f; f = /**@nosideeffects*/function(){};", "var a = f();",
"", null, null);
}
public void testNoSideEffectAnnotation7() {
test("var f;" +
"f = /**@nosideeffects*/function(){};",
"f = function(){};" +
"var a = f();",
"f = function(){}; f();", null, null);
}
public void testNoSideEffectAnnotation8() {
test("var f;" +
"f = function(){};" +
"f = /**@nosideeffects*/function(){};",
"var a = f();",
"f();", null, null);
}
public void testNoSideEffectAnnotation9() {
test("var f;" +
"f = /**@nosideeffects*/function(){};" +
"f = /**@nosideeffects*/function(){};",
"var a = f();",
"", null, null);
test("var f; f = /**@nosideeffects*/function(){};", "var a = f();",
"", null, null);
}
public void testNoSideEffectAnnotation10() {
test("var o = {}; o.f = function(){}; var a = o.f();",
"var o = {}; o.f = function(){}; o.f();");
}
public void testNoSideEffectAnnotation11() {
test("var o = {}; o.f = /**@nosideeffects*/function(){};",
"var a = o.f();", "", null, null);
}
public void testNoSideEffectAnnotation12() {
test("function c(){} var a = new c",
"function c(){} new c");
}
public void testNoSideEffectAnnotation13() {
test("/**@nosideeffects*/function c(){}", "var a = new c",
"", null, null);
}
public void testNoSideEffectAnnotation14() {
String common = "function c(){};" +
"c.prototype.f = /**@nosideeffects*/function(){};";
test(common, "var o = new c; var a = o.f()", "new c", null, null);
}
public void testNoSideEffectAnnotation15() {
test("function c(){}; c.prototype.f = function(){}; var a = (new c).f()",
"function c(){}; c.prototype.f = function(){}; (new c).f()");
}
public void testNoSideEffectAnnotation16() {
test("/**@nosideeffects*/function c(){}" +
"c.prototype.f = /**@nosideeffects*/function(){};",
"var a = (new c).f()",
"",
null, null);
}
public void testFunctionPrototype() {
testSame("var a = 5; Function.prototype.foo = function() {return a;}");
}
public void testTopLevelClass1() {
test("var Point = function() {}; Point.prototype.foo = function() {}", "");
}
public void testTopLevelClass2() {
testSame("var Point = {}; Point.prototype.foo = function() {};" +
"externfoo = new Point()");
}
public void testTopLevelClass3() {
test("function Point() {this.me_ = Point}", "");
}
public void testTopLevelClass4() {
test("function f(){} function A(){} A.prototype = {x: function() {}}; f();",
"function f(){} f();");
}
public void testTopLevelClass5() {
testSame("function f(){} function A(){}" +
"A.prototype = {x: function() { f(); }}; new A();");
}
public void testTopLevelClass6() {
testSame("function f(){} function A(){}" +
"A.prototype = {x: function() { f(); }}; new A().x();");
}
public void testTopLevelClass7() {
test("A.prototype.foo = function(){}; function A() {}", "");
}
public void testNamespacedClass1() {
test("var foo = {};foo.bar = {};foo.bar.prototype.baz = {}", "");
}
public void testNamespacedClass2() {
testSame("var foo = {};foo.bar = {};foo.bar.prototype.baz = {};" +
"window.z = new foo.bar()");
}
public void testNamespacedClass3() {
test("var a = {}; a.b = function() {}; a.b.prototype = {x: function() {}};",
"");
}
public void testNamespacedClass4() {
testSame("function f(){} var a = {}; a.b = function() {};" +
"a.b.prototype = {x: function() { f(); }}; new a.b();");
}
public void testNamespacedClass5() {
testSame("function f(){} var a = {}; a.b = function() {};" +
"a.b.prototype = {x: function() { f(); }}; new a.b().x();");
}
public void testAssignmentToThisPrototype() {
testSame("Function.prototype.inherits = function(parentCtor) {" +
" function tempCtor() {};" +
" tempCtor.prototype = parentCtor.prototype;" +
" this.superClass_ = parentCtor.prototype;" +
" this.prototype = new tempCtor();" +
" this.prototype.constructor = this;" +
"};");
}
public void testAssignmentToCallResultPrototype() {
testSame("function f() { return function(){}; } f().prototype = {};");
}
public void testAssignmentToExternPrototype() {
testSame("externfoo.prototype = {};");
}
public void testAssignmentToUnknownPrototype() {
testSame(
"/** @suppress {duplicate} */ var window;" +
"window['a'].prototype = {};");
}
public void testBug2099540() {
testSame(
"/** @suppress {duplicate} */ var document;\n" +
"/** @suppress {duplicate} */ var window;\n" +
"var klass;\n" +
"window[klass].prototype = " +
"document.createElement(tagName)['__proto__'];");
}
public void testOtherGlobal() {
testSame("goog.global.foo = bar(); function bar(){}");
}
public void testExternName1() {
testSame("top.z = bar(); function bar(){}");
}
public void testExternName2() {
testSame("top['z'] = bar(); function bar(){}");
}
public void testInherits1() {
test("var a = {}; var b = {}; b.inherits(a)", "");
}
public void testInherits2() {
test("var a = {}; var b = {}; var goog = {}; goog.inherits(b, a)", "");
}
public void testInherits3() {
testSame("var a = {}; this.b = {}; b.inherits(a);");
}
public void testInherits4() {
testSame("var a = {}; this.b = {}; var goog = {}; goog.inherits(b, a);");
}
public void testInherits5() {
test("this.a = {}; var b = {}; b.inherits(a);",
"this.a = {}");
}
public void testInherits6() {
test("this.a = {}; var b = {}; var goog = {}; goog.inherits(b, a);",
"this.a = {}");
}
public void testInherits7() {
testSame("var a = {}; this.b = {}; var goog = {};" +
" goog.inherits = function() {}; goog.inherits(b, a);");
}
public void testInherits8() {
// Make sure that exceptions aren't thrown if inherits() is used as
// an R-value
test("this.a = {}; var b = {}; var c = b.inherits(a);", "this.a = {};");
}
public void testMixin1() {
testSame("var goog = {}; goog.mixin = function() {};" +
"Function.prototype.mixin = function(base) {" +
" goog.mixin(this.prototype, base); " +
"};");
}
public void testMixin2() {
testSame("var a = {}; this.b = {}; var goog = {};" +
" goog.mixin = function() {}; goog.mixin(b.prototype, a.prototype);");
}
public void testMixin3() {
test("this.a = {}; var b = {}; var goog = {};" +
" goog.mixin = function() {}; goog.mixin(b.prototype, a.prototype);",
"this.a = {};");
}
public void testMixin4() {
testSame("this.a = {}; var b = {}; var goog = {};" +
"goog.mixin = function() {};" +
"goog.mixin(b.prototype, a.prototype);" +
"new b()");
}
public void testMixin5() {
test("this.a = {}; var b = {}; var c = {}; var goog = {};" +
"goog.mixin = function() {};" +
"goog.mixin(b.prototype, a.prototype);" +
"goog.mixin(c.prototype, a.prototype);" +
"new b()",
"this.a = {}; var b = {}; var goog = {};" +
"goog.mixin = function() {};" +
"goog.mixin(b.prototype, a.prototype);" +
"new b()");
}
public void testMixin6() {
testSame("this.a = {}; var b = {}; var c = {}; var goog = {};" +
"goog.mixin = function() {};" +
"goog.mixin(c.prototype, a.prototype) + " +
"goog.mixin(b.prototype, a.prototype);" +
"new b()");
}
public void testMixin7() {
test("this.a = {}; var b = {}; var c = {}; var goog = {};" +
"goog.mixin = function() {};" +
"var d = goog.mixin(c.prototype, a.prototype) + " +
"goog.mixin(b.prototype, a.prototype);" +
"new b()",
"this.a = {}; var b = {}; var goog = {};" +
"goog.mixin = function() {};" +
"goog.mixin(b.prototype, a.prototype);" +
"new b()");
}
public void testConstants1() {
testSame("var bar = function(){}; var EXP_FOO = true; if (EXP_FOO) bar();");
}
public void testConstants2() {
test("var bar = function(){}; var EXP_FOO = true; var EXP_BAR = true;" +
"if (EXP_FOO) bar();",
"var bar = function(){}; var EXP_FOO = true; if (EXP_FOO) bar();");
}
public void testExpressions1() {
test("var foo={}; foo.A='A'; foo.AB=foo.A+'B'; foo.ABC=foo.AB+'C'",
"");
}
public void testExpressions2() {
testSame("var foo={}; foo.A='A'; foo.AB=foo.A+'B'; this.ABC=foo.AB+'C'");
}
public void testExpressions3() {
testSame("var foo = 2; window.bar(foo + 3)");
}
public void testSetCreatingReference() {
testSame("var foo; var bar = function(){foo=6;}; bar();");
}
public void testAnonymous1() {
testSame("function foo() {}; function bar() {}; foo(function() {bar()})");
}
public void testAnonymous2() {
test("var foo;(function(){foo=6;})()", "(function(){})()");
}
public void testAnonymous3() {
testSame("var foo; (function(){ if(!foo)foo=6; })()");
}
public void testAnonymous4() {
testSame("var foo; (function(){ foo=6; })(); externfoo=foo;");
}
public void testAnonymous5() {
testSame("var foo;" +
"(function(){ foo=function(){ bar() }; function bar(){} })();" +
"foo();");
}
public void testAnonymous6() {
testSame("function foo(){}" +
"function bar(){}" +
"foo(function(){externfoo = bar});");
}
public void testAnonymous7() {
testSame("var foo;" +
"(function (){ function bar(){ externfoo = foo; } bar(); })();");
}
public void testAnonymous8() {
testSame("var foo;" +
"(function (){ var g=function(){ externfoo = foo; }; g(); })();");
}
public void testAnonymous9() {
testSame("function foo(){}" +
"function bar(){}" +
"foo(function(){ function baz(){ externfoo = bar; } baz(); });");
}
public void testFunctions1() {
testSame("var foo = null; function baz() {}" +
"function bar() {foo=baz();} bar();");
}
public void testFunctions2() {
testSame("var foo; foo = function() {var a = bar()};" +
"var bar = function(){}; foo();");
}
public void testGetElem1() {
testSame("var foo = {}; foo.bar = {}; foo.bar.baz = {a: 5, b: 10};" +
"var fn = function() {window[foo.bar.baz.a] = 5;}; fn()");
}
public void testGetElem2() {
testSame("var foo = {}; foo.bar = {}; foo.bar.baz = {a: 5, b: 10};" +
"var fn = function() {this[foo.bar.baz.a] = 5;}; fn()");
}
public void testGetElem3() {
testSame("var foo = {'i': 0, 'j': 1}; foo['k'] = 2; top.foo = foo;");
}
public void testIf1() {
test("var foo = {};if(e)foo.bar=function(){};", "if(e);");
}
public void testIf2() {
test("var e = false;var foo = {};if(e)foo.bar=function(){};",
"var e = false;if(e);");
}
public void testIf3() {
test("var e = false;var foo = {};if(e + 1)foo.bar=function(){};",
"var e = false;if(e + 1);");
}
public void testIf4() {
test("var e = false, f;var foo = {};if(f=e)foo.bar=function(){};",
"var e = false;if(e);");
}
public void testIf4a() {
// TODO(johnlenz): fix this.
testSame("var e = [], f;if(f=e);f[0] = 1;");
}
public void testIf4b() {
// TODO(johnlenz): fix this.
test("var e = [], f;if(e=f);f[0] = 1;",
"var f;if(f);f[0] = 1;");
}
public void testIf4c() {
test("var e = [], f;if(f=e);e[0] = 1;",
"var e = [];if(e);e[0] = 1;");
}
public void testIf5() {
test("var e = false, f;var foo = {};if(f = e + 1)foo.bar=function(){};",
"var e = false;if(e + 1);");
}
public void testIfElse() {
test("var foo = {};if(e)foo.bar=function(){};else foo.bar=function(){};",
"if(e);else;");
}
public void testWhile() {
test("var foo = {};while(e)foo.bar=function(){};", "while(e);");
}
public void testFor() {
test("var foo = {};for(e in x)foo.bar=function(){};", "for(e in x);");
}
public void testDo() {
test("var cond = false;do {var a = 1} while (cond)",
"var cond = false;do {} while (cond)");
}
public void testSetterInForStruct1() {
test("var j = 0; for (var i = 1; i = 0; j++);",
"var j = 0; for (; 0; j++);");
}
public void testSetterInForStruct2() {
test("var Class = function() {}; " +
"for (var i = 1; Class.prototype.property_ = 0; i++);",
"for (var i = 1; 0; i++);");
}
public void testSetterInForStruct3() {
test("var j = 0; for (var i = 1 + f() + g() + h(); i = 0; j++);",
"var j = 0; f(); g(); h(); for (; 0; j++);");
}
public void testSetterInForStruct4() {
test("var i = 0;var j = 0; for (i = 1 + f() + g() + h(); i = 0; j++);",
"var j = 0; f(); g(); h(); for (; 0; j++);");
}
public void testSetterInForStruct5() {
test("var i = 0, j = 0; for (i = f(), j = g(); 0;);",
"for (f(), g(); 0;);");
}
public void testSetterInForStruct6() {
test("var i = 0, j = 0, k = 0; for (i = f(), j = g(), k = h(); i = 0;);",
"for (f(), g(), h(); 0;);");
}
public void testSetterInForStruct7() {
test("var i = 0, j = 0, k = 0; for (i = 1, j = 2, k = 3; i = 0;);",
"for (1, 2, 3; 0;);");
}
public void testSetterInForStruct8() {
test("var i = 0, j = 0, k = 0; for (i = 1, j = i, k = 2; i = 0;);",
"var i = 0; for(i = 1, i , 2; i = 0;);");
}
public void testSetterInForStruct9() {
test("var Class = function() {}; " +
"for (var i = 1; Class.property_ = 0; i++);",
"for (var i = 1; 0; i++);");
}
public void testSetterInForStruct10() {
test("var Class = function() {}; " +
"for (var i = 1; Class.property_ = 0; i = 2);",
"for (; 0;);");
}
public void testSetterInForStruct11() {
test("var Class = function() {}; " +
"for (;Class.property_ = 0;);",
"for (;0;);");
}
public void testSetterInForStruct12() {
test("var a = 1; var Class = function() {}; " +
"for (;Class.property_ = a;);",
"var a = 1; for (; a;);");
}
public void testSetterInForStruct13() {
test("var a = 1; var Class = function() {}; " +
"for (Class.property_ = a; 0 ;);",
"for (; 0;);");
}
public void testSetterInForStruct14() {
test("var a = 1; var Class = function() {}; " +
"for (; 0; Class.property_ = a);",
"for (; 0;);");
}
public void testSetterInForStruct15() {
test("var Class = function() {}; " +
"for (var i = 1; 0; Class.prototype.property_ = 0);",
"for (; 0; 0);");
}
public void testSetterInForStruct16() {
test("var Class = function() {}; " +
"for (var i = 1; i = 0; Class.prototype.property_ = 0);",
"for (; 0; 0);");
}
public void testSetterInForIn1() {
test("var foo = {}; var bar; for(e in bar = foo.a);",
"var foo = {}; for(e in foo.a);");
}
public void testSetterInForIn2() {
testSame("var foo = {}; var bar; for(e in bar = foo.a); bar");
}
public void testSetterInForIn3() {
testSame("var foo = {}; var bar; for(e in bar = foo.a); bar.b = 3");
}
public void testSetterInForIn4() {
testSame("var foo = {}; var bar; for (e in bar = foo.a); bar.b = 3; foo.a");
}
public void testSetterInForIn5() {
// TODO(user) Fix issue similar to b/2316773: bar should be preserved
// but isn't due to missing references between e and foo.a
test("var foo = {}; var bar; for (e in foo.a) { bar = e } bar.b = 3; foo.a",
"var foo={};for(e in foo.a);foo.a");
}
public void testSetterInForIn6() {
testSame("var foo = {};for(e in foo);");
}
public void testSetterInIfPredicate() {
// TODO(user) Make NameAnalyzer smarter so it can remove "Class".
testSame("var a = 1;" +
"var Class = function() {}; " +
"if (Class.property_ = a);");
}
public void testSetterInWhilePredicate() {
test("var a = 1;" +
"var Class = function() {}; " +
"while (Class.property_ = a);",
"var a = 1; for (;a;) {}");
}
public void testSetterInDoWhilePredicate() {
// TODO(user) Make NameAnalyzer smarter so it can remove "Class".
testSame("var a = 1;" +
"var Class = function() {}; " +
"do {} while(Class.property_ = a);");
}
public void testSetterInSwitchInput() {
// TODO(user) Make NameAnalyzer smarter so it can remove "Class".
testSame("var a = 1;" +
"var Class = function() {}; " +
"switch (Class.property_ = a) {" +
" default:" +
"}");
}
public void testComplexAssigns() {
// Complex assigns are not removed by the current pass.
testSame("var x = 0; x += 3; x *= 5;");
}
public void testNestedAssigns1() {
test("var x = 0; var y = x = 3; window.alert(y);",
"var y = 3; window.alert(y);");
}
public void testNestedAssigns2() {
testSame("var x = 0; var y = x = {}; x.b = 3; window.alert(y);");
}
public void testComplexNestedAssigns1() {
// TODO(nicksantos): Make NameAnalyzer smarter, so that we can eliminate y.
testSame("var x = 0; var y = 2; y += x = 3; window.alert(x);");
}
public void testComplexNestedAssigns2() {
test("var x = 0; var y = 2; y += x = 3; window.alert(y);",
"var y = 2; y += 3; window.alert(y);");
}
public void testComplexNestedAssigns3() {
test("var x = 0; var y = x += 3; window.alert(x);",
"var x = 0; x += 3; window.alert(x);");
}
public void testComplexNestedAssigns4() {
testSame("var x = 0; var y = x += 3; window.alert(y);");
}
public void testUnintendedUseOfInheritsInLocalScope1() {
testSame("goog.mixin = function() {}; " +
"(function() { var x = {}; var y = {}; goog.mixin(x, y); })();");
}
public void testUnintendedUseOfInheritsInLocalScope2() {
testSame("goog.mixin = function() {}; " +
"var x = {}; var y = {}; (function() { goog.mixin(x, y); })();");
}
public void testUnintendedUseOfInheritsInLocalScope3() {
testSame("goog.mixin = function() {}; " +
"var x = {}; var y = {}; (function() { goog.mixin(x, y); })(); " +
"window.alert(x);");
}
public void testUnintendedUseOfInheritsInLocalScope4() {
// Ensures that the "goog$mixin" variable doesn't get stripped out,
// even when it's only used in a local scope.
testSame("var goog$mixin = function() {}; " +
"(function() { var x = {}; var y = {}; goog$mixin(x, y); })();");
}
public void testPrototypePropertySetInLocalScope1() {
testSame("(function() { var x = function(){}; x.prototype.bar = 3; })();");
}
public void testPrototypePropertySetInLocalScope2() {
testSame("var x = function(){}; (function() { x.prototype.bar = 3; })();");
}
public void testPrototypePropertySetInLocalScope3() {
test("var x = function(){ x.prototype.bar = 3; };", "");
}
public void testPrototypePropertySetInLocalScope4() {
test("var x = {}; x.foo = function(){ x.foo.prototype.bar = 3; };", "");
}
public void testPrototypePropertySetInLocalScope5() {
test("var x = {}; x.prototype.foo = 3;", "");
}
public void testPrototypePropertySetInLocalScope6() {
testSame("var x = {}; x.prototype.foo = 3; bar(x.prototype.foo)");
}
public void testPrototypePropertySetInLocalScope7() {
testSame("var x = {}; x.foo = 3; bar(x.foo)");
}
public void testRValueReference1() {
testSame("var a = 1; a");
}
public void testRValueReference2() {
testSame("var a = 1; 1+a");
}
public void testRValueReference3() {
testSame("var x = {}; x.prototype.foo = 3; var a = x.prototype.foo; 1+a");
}
public void testRValueReference4() {
testSame("var x = {}; x.prototype.foo = 3; x.prototype.foo");
}
public void testRValueReference5() {
testSame("var x = {}; x.prototype.foo = 3; 1+x.prototype.foo");
}
public void testRValueReference6() {
testSame("var x = {}; var idx = 2; x[idx]");
}
public void testUnhandledTopNode() {
testSame("function Foo() {}; Foo.prototype.isBar = function() {};" +
"function Bar() {}; Bar.prototype.isFoo = function() {};" +
"var foo = new Foo(); var bar = new Bar();" +
// The boolean AND here is currently unhandled by this pass, but
// it should not cause it to blow up.
"var cond = foo.isBar() && bar.isFoo();" +
"if (cond) {window.alert('hello');}");
}
public void testPropertyDefinedInGlobalScope() {
testSame("function Foo() {}; var x = new Foo(); x.cssClass = 'bar';" +
"window.alert(x);");
}
public void testConditionallyDefinedFunction1() {
testSame("var g; externfoo.x || (externfoo.x = function() { g; })");
}
public void testConditionallyDefinedFunction2() {
testSame("var g; 1 || (externfoo.x = function() { g; })");
}
public void testConditionallyDefinedFunction3() {
testSame("var a = {};" +
"rand() % 2 || (a.f = function() { externfoo = 1; } || alert());");
}
public void testGetElemOnThis() {
testSame("var a = 3; this['foo'] = a;");
testSame("this['foo'] = 3;");
}
public void testRemoveInstanceOfOnly() {
test("function Foo() {}; Foo.prototype.isBar = function() {};" +
"var x; if (x instanceof Foo) { window.alert(x); }",
";var x; if (false) { window.alert(x); }");
}
public void testRemoveLocalScopedInstanceOfOnly() {
test("function Foo() {}; function Bar(x) { this.z = x instanceof Foo; };" +
"externfoo.x = new Bar({});",
";function Bar(x) { this.z = false }; externfoo.x = new Bar({});");
}
public void testRemoveInstanceOfWithReferencedMethod() {
test("function Foo() {}; Foo.prototype.isBar = function() {};" +
"var x; if (x instanceof Foo) { window.alert(x.isBar()); }",
";var x; if (false) { window.alert(x.isBar()); }");
}
public void testDoNotChangeReferencedInstanceOf() {
testSame("function Foo() {}; Foo.prototype.isBar = function() {};" +
"var x = new Foo(); if (x instanceof Foo) { window.alert(x); }");
}
public void testDoNotChangeReferencedLocalScopedInstanceOf() {
testSame("function Foo() {}; externfoo.x = new Foo();" +
"function Bar() { if (x instanceof Foo) { window.alert(x); } };" +
"externfoo.y = new Bar();");
}
public void testDoNotChangeLocalScopeReferencedInstanceOf() {
testSame("function Foo() {}; Foo.prototype.isBar = function() {};" +
"function Bar() { this.z = new Foo(); }; externfoo.x = new Bar();" +
"if (x instanceof Foo) { window.alert(x); }");
}
public void testDoNotChangeLocalScopeReferencedLocalScopedInstanceOf() {
testSame("function Foo() {}; Foo.prototype.isBar = function() {};" +
"function Bar() { this.z = new Foo(); };" +
"Bar.prototype.func = function(x) {" +
"if (x instanceof Foo) { window.alert(x); }" +
"}; new Bar().func();");
}
public void testDoNotChangeLocalScopeReferencedLocalScopedInstanceOf2() {
test(
"function Foo() {}" +
"var createAxis = function(f) { return window.passThru(f); };" +
"var axis = createAxis(function(test) {" +
" return test instanceof Foo;" +
"});",
"var createAxis = function(f) { return window.passThru(f); };" +
"createAxis(function(test) {" +
" return false;" +
"});");
}
public void testDoNotChangeInstanceOfGetElem() {
testSame("var goog = {};" +
"function f(obj, name) {" +
" if (obj instanceof goog[name]) {" +
" return name;" +
" }" +
"}" +
"window['f'] = f;");
}
public void testWeirdnessOnLeftSideOfPrototype() {
// This checks a bug where 'x' was removed, but the function referencing
// it was not, causing problems.
testSame("var x = 3; " +
"(function() { this.bar = 3; }).z = function() {" +
" return x;" +
"};");
}
public void testShortCircuit1() {
test("var a = b() || 1", "b()");
}
public void testShortCircuit2() {
test("var a = 1 || c()", "1 || c()");
}
public void testShortCircuit3() {
test("var a = b() || c()", "b() || c()");
}
public void testShortCircuit4() {
test("var a = b() || 3 || c()", "b() || 3 || c()");
}
public void testShortCircuit5() {
test("var a = b() && 1", "b()");
}
public void testShortCircuit6() {
test("var a = 1 && c()", "1 && c()");
}
public void testShortCircuit7() {
test("var a = b() && c()", "b() && c()");
}
public void testShortCircuit8() {
test("var a = b() && 3 && c()", "b() && 3 && c()");
}
public void testRhsReference1() {
testSame("var a = 1; a");
}
public void testRhsReference2() {
testSame("var a = 1; a || b()");
}
public void testRhsReference3() {
testSame("var a = 1; 1 || a");
}
public void testRhsReference4() {
test("var a = 1; var b = a || foo()", "var a = 1; a || foo()");
}
public void testRhsReference5() {
test("var a = 1, b = 5; a; foo(b)", "var a = 1, b = 5; a; foo(b)");
}
public void testRhsAssign1() {
test("var foo, bar; foo || (bar = 1)",
"var foo; foo || 1");
}
public void testRhsAssign2() {
test("var foo, bar, baz; foo || (baz = bar = 1)",
"var foo; foo || 1");
}
public void testRhsAssign3() {
testSame("var foo = null; foo || (foo = 1)");
}
public void testRhsAssign4() {
test("var foo = null; foo = (foo || 1)", "");
}
public void testRhsAssign5() {
test("var a = 3, foo, bar; foo || (bar = a)", "var a = 3, foo; foo || a");
}
public void testRhsAssign6() {
test("function Foo(){} var foo = null;" +
"var f = function () {foo || (foo = new Foo()); return foo}",
"");
}
public void testRhsAssign7() {
testSame("function Foo(){} var foo = null;" +
"var f = function () {foo || (foo = new Foo())}; f()");
}
public void testRhsAssign8() {
testSame("function Foo(){} var foo = null;" +
"var f = function () {(foo = new Foo()) || g()}; f()");
}
public void testRhsAssign9() {
test("function Foo(){} var foo = null;" +
"var f = function () {1 + (foo = new Foo()); return foo}",
"");
}
public void testAssignWithOr1() {
testSame("var foo = null;" +
"var f = window.a || function () {return foo}; f()");
}
public void testAssignWithOr2() {
test("var foo = null;" +
"var f = window.a || function () {return foo};",
"var foo = null"); // why is this left?
}
public void testAssignWithAnd1() {
testSame("var foo = null;" +
"var f = window.a && function () {return foo}; f()");
}
public void testAssignWithAnd2() {
test("var foo = null;" +
"var f = window.a && function () {return foo};",
"var foo = null;"); // why is this left?
}
public void testAssignWithHook1() {
testSame("function Foo(){} var foo = null;" +
"var f = window.a ? " +
" function () {return new Foo()} : function () {return foo}; f()");
}
public void testAssignWithHook2() {
test("function Foo(){} var foo = null;" +
"var f = window.a ? " +
" function () {return new Foo()} : function () {return foo};",
"");
}
public void testAssignWithHook2a() {
test("function Foo(){} var foo = null;" +
"var f; f = window.a ? " +
" function () {return new Foo()} : function () {return foo};",
"");
}
public void testAssignWithHook3() {
testSame("function Foo(){} var foo = null; var f = {};" +
"f.b = window.a ? " +
" function () {return new Foo()} : function () {return foo}; f.b()");
}
public void testAssignWithHook4() {
test("function Foo(){} var foo = null; var f = {};" +
"f.b = window.a ? " +
" function () {return new Foo()} : function () {return foo};",
"");
}
public void testAssignWithHook5() {
testSame("function Foo(){} var foo = null; var f = {};" +
"f.b = window.a ? function () {return new Foo()} :" +
" window.b ? function () {return foo} :" +
" function() { return Foo }; f.b()");
}
public void testAssignWithHook6() {
test("function Foo(){} var foo = null; var f = {};" +
"f.b = window.a ? function () {return new Foo()} :" +
" window.b ? function () {return foo} :" +
" function() { return Foo };",
"");
}
public void testAssignWithHook7() {
testSame("function Foo(){} var foo = null;" +
"var f = window.a ? new Foo() : foo;" +
"f()");
}
public void testAssignWithHook8() {
test("function Foo(){} var foo = null;" +
"var f = window.a ? new Foo() : foo;",
"function Foo(){}" +
"window.a && new Foo()");
}
public void testAssignWithHook9() {
test("function Foo(){} var foo = null; var f = {};" +
"f.b = window.a ? new Foo() : foo;",
"function Foo(){} window.a && new Foo()");
}
public void testAssign1() {
test("function Foo(){} var foo = null; var f = {};" +
"f.b = window.a;",
"");
}
public void testAssign2() {
test("function Foo(){} var foo = null; var f = {};" +
"f.b = window;",
"");
}
public void testAssign3() {
test("var f = {};" +
"f.b = window;",
"");
}
public void testAssign4() {
test("function Foo(){} var foo = null; var f = {};" +
"f.b = new Foo();",
"function Foo(){} new Foo()");
}
public void testAssign5() {
test("function Foo(){} var foo = null; var f = {};" +
"f.b = foo;",
"");
}
public void testAssignWithCall() {
test("var fun, x; (fun = function(){ x; })();",
"var x; (function(){ x; })();");
}
// Currently this crashes the compiler because it erroneoursly removes var x
// and later a sanity check fails.
public void testAssignWithCall2() {
test("var fun, x; (123, fun = function(){ x; })();",
"(123, function(){ x; })();");
}
public void testNestedAssign1() {
test("var a, b = a = 1, c = 2", "");
}
public void testNestedAssign2() {
test("var a, b = a = 1; foo(b)",
"var b = 1; foo(b)");
}
public void testNestedAssign3() {
test("var a, b = a = 1; a = b = 2; foo(b)",
"var b = 1; b = 2; foo(b)");
}
public void testNestedAssign4() {
test("var a, b = a = 1; b = a = 2; foo(b)",
"var b = 1; b = 2; foo(b)");
}
public void testNestedAssign5() {
test("var a, b = a = 1; b = a = 2", "");
}
public void testNestedAssign15() {
test("var a, b, c; c = b = a = 2", "");
}
public void testNestedAssign6() {
testSame("var a, b, c; a = b = c = 1; foo(a, b, c)");
}
public void testNestedAssign7() {
testSame("var a = 0; a = i[j] = 1; b(a, i[j])");
}
public void testNestedAssign8() {
testSame("function f(){" +
"this.lockedToken_ = this.lastToken_ = " +
"SETPROP_value(this.hiddenInput_, a)}f()");
}
public void testRefChain1() {
test("var a = 1; var b = a; var c = b; var d = c", "");
}
public void testRefChain2() {
test("var a = 1; var b = a; var c = b; var d = c || f()",
"var a = 1; var b = a; var c = b; c || f()");
}
public void testRefChain3() {
test("var a = 1; var b = a; var c = b; var d = c + f()", "f()");
}
public void testRefChain4() {
test("var a = 1; var b = a; var c = b; var d = f() || c",
"f()");
}
public void testRefChain5() {
test("var a = 1; var b = a; var c = b; var d = f() ? g() : c",
"f() && g()");
}
public void testRefChain6() {
test("var a = 1; var b = a; var c = b; var d = c ? f() : g()",
"var a = 1; var b = a; var c = b; c ? f() : g()");
}
public void testRefChain7() {
test("var a = 1; var b = a; var c = b; var d = (b + f()) ? g() : c",
"var a = 1; var b = a; (b+f()) && g()");
}
public void testRefChain8() {
test("var a = 1; var b = a; var c = b; var d = f()[b] ? g() : 0",
"var a = 1; var b = a; f()[b] && g()");
}
public void testRefChain9() {
test("var a = 1; var b = a; var c = 5; var d = f()[b+c] ? g() : 0",
"var a = 1; var b = a; var c = 5; f()[b+c] && g()");
}
public void testRefChain10() {
test("var a = 1; var b = a; var c = b; var d = f()[b] ? g() : 0",
"var a = 1; var b = a; f()[b] && g()");
}
public void testRefChain11() {
test("var a = 1; var b = a; var d = f()[b] ? g() : 0",
"var a = 1; var b = a; f()[b] && g()");
}
public void testRefChain12() {
testSame("var a = 1; var b = a; f()[b] ? g() : 0");
}
public void testRefChain13() {
test("function f(){}var a = 1; var b = a; var d = f()[b] ? g() : 0",
"function f(){}var a = 1; var b = a; f()[b] && g()");
}
public void testRefChain14() {
testSame("function f(){}var a = 1; var b = a; f()[b] ? g() : 0");
}
public void testRefChain15() {
test("function f(){}var a = 1, b = a; var c = f(); var d = c[b] ? g() : 0",
"function f(){}var a = 1, b = a; var c = f(); c[b] && g()");
}
public void testRefChain16() {
testSame("function f(){}var a = 1; var b = a; var c = f(); c[b] ? g() : 0");
}
public void testRefChain17() {
test("function f(){}var a = 1; var b = a; var c = f(); var d = c[b]",
"function f(){} f()");
}
public void testRefChain18() {
testSame("var a = 1; f()[a] && g()");
}
public void testRefChain19() {
test("var a = 1; var b = [a]; var c = b; b[f()] ? g() : 0",
"var a=1; var b=[a]; b[f()] ? g() : 0");
}
public void testRefChain20() {
test("var a = 1; var b = [a]; var c = b; var d = b[f()] ? g() : 0",
"var a=1; var b=[a]; b[f()]&&g()");
}
public void testRefChain21() {
testSame("var a = 1; var b = 2; var c = a + b; f(c)");
}
public void testRefChain22() {
test("var a = 2; var b = a = 4; f(a)", "var a = 2; a = 4; f(a)");
}
public void testRefChain23() {
test("var a = {}; var b = a[1] || f()", "var a = {}; a[1] || f()");
}
/**
* Expressions that cannot be attributed to any enclosing dependency
* scope should be treated as global references.
* @bug 1739062
*/
public void testAssignmentWithComplexLhs() {
testSame("function f() { return this; }" +
"var o = {'key': 'val'};" +
"f().x_ = o['key'];");
}
public void testAssignmentWithComplexLhs2() {
testSame("function f() { return this; }" +
"var o = {'key': 'val'};" +
"f().foo = function() {" +
" o" +
"};");
}
public void testAssignmentWithComplexLhs3() {
String source =
"var o = {'key': 'val'};" +
"function init_() {" +
" this.x = o['key']" +
"}";
test(source, "");
testSame(source + ";init_()");
}
public void testAssignmentWithComplexLhs4() {
testSame("function f() { return this; }" +
"var o = {'key': 'val'};" +
"f().foo = function() {" +
" this.x = o['key']" +
"};");
}
/**
* Do not "prototype" property of variables that are not being
* tracked (because they are local).
* @bug 1809442
*/
public void testNoRemovePrototypeDefinitionsOutsideGlobalScope1() {
testSame("function f(arg){}" +
"" +
"(function(){" +
" var O = {};" +
" O.prototype = 'foo';" +
" f(O);" +
"})()");
}
public void testNoRemovePrototypeDefinitionsOutsideGlobalScope2() {
testSame("function f(arg){}" +
"(function h(){" +
" var L = {};" +
" L.prototype = 'foo';" +
" f(L);" +
"})()");
}
public void testNoRemovePrototypeDefinitionsOutsideGlobalScope4() {
testSame("function f(arg){}" +
"function g(){" +
" var N = {};" +
" N.prototype = 'foo';" +
" f(N);" +
"}" +
"g()");
}
public void testNoRemovePrototypeDefinitionsOutsideGlobalScope5() {
// function body not removed due to @bug 1898561
testSame("function g(){ var R = {}; R.prototype = 'foo' } g()");
}
public void testRemovePrototypeDefinitionsInGlobalScope1() {
testSame("function f(arg){}" +
"var M = {};" +
"M.prototype = 'foo';" +
"f(M);");
}
public void testRemovePrototypeDefinitionsInGlobalScope2() {
test("var Q = {}; Q.prototype = 'foo'", "");
}
public void testRemoveLabeledStatment() {
test("LBL: var x = 1;", "LBL: {}");
}
public void testRemoveLabeledStatment2() {
test("var x; LBL: x = f() + g()", "LBL: { f() ; g()}");
}
public void testRemoveLabeledStatment3() {
test("var x; LBL: x = 1;", "LBL: {}");
}
public void testRemoveLabeledStatment4() {
test("var a; LBL: a = f()", "LBL: f()");
}
public void testPreservePropertyMutationsToAlias1() {
// Test for issue b/2316773 - property get case
// Since a is referenced, property mutations via a's alias b must
// be preserved.
testSame("var a = {}; var b = a; b.x = 1; a");
}
public void testPreservePropertyMutationsToAlias2() {
// Test for issue b/2316773 - property get case, don't keep 'c'
test("var a = {}; var b = a; var c = a; b.x = 1; a",
"var a = {}; var b = a; b.x = 1; a");
}
public void testPreservePropertyMutationsToAlias3() {
// Test for issue b/2316773 - property get case, chain
testSame("var a = {}; var b = a; var c = b; c.x = 1; a");
}
public void testPreservePropertyMutationsToAlias4() {
// Test for issue b/2316773 - element get case
testSame("var a = {}; var b = a; b['x'] = 1; a");
}
public void testPreservePropertyMutationsToAlias5() {
// From issue b/2316773 description
testSame("function testCall(o){}" +
"var DATA = {'prop': 'foo','attr': {}};" +
"var SUBDATA = DATA['attr'];" +
"SUBDATA['subprop'] = 'bar';" +
"testCall(DATA);");
}
public void testPreservePropertyMutationsToAlias6() {
// Longer GETELEM chain
testSame("function testCall(o){}" +
"var DATA = {'prop': 'foo','attr': {}};" +
"var SUBDATA = DATA['attr'];" +
"var SUBSUBDATA = SUBDATA['subprop'];" +
"SUBSUBDATA['subsubprop'] = 'bar';" +
"testCall(DATA);");
}
public void testPreservePropertyMutationsToAlias7() {
// Make sure that the base class does not depend on the derived class.
test("var a = {}; var b = {}; b.x = 0;" +
"var goog = {}; goog.inherits(b, a); a",
"var a = {}; a");
}
public void testPreservePropertyMutationsToAlias8() {
// Make sure that the derived classes don't end up depending on each other.
test("var a = {};" +
"var b = {}; b.x = 0;" +
"var c = {}; c.y = 0;" +
"var goog = {}; goog.inherits(b, a); goog.inherits(c, a); c",
"var a = {}; var c = {}; c.y = 0;" +
"var goog = {}; goog.inherits(c, a); c");
}
public void testPreservePropertyMutationsToAlias9() {
testSame("var a = {b: {}};" +
"var c = a.b; c.d = 3;" +
"a.d = 3; a.d;");
}
public void testRemoveAlias() {
test("var a = {b: {}};" +
"var c = a.b;" +
"a.d = 3; a.d;",
"var a = {b: {}}; a.d = 3; a.d;");
}
public void testSingletonGetter1() {
test("function Foo() {} goog.addSingletonGetter(Foo);", "");
}
public void testSingletonGetter2() {
test("function Foo() {} goog$addSingletonGetter(Foo);", "");
}
public void testSingletonGetter3() {
// addSingletonGetter adds a getInstance method to a class.
testSame("function Foo() {} goog$addSingletonGetter(Foo);" +
"this.x = Foo.getInstance();");
}
public void testNoRemoveWindowPropertyAlias1() {
testSame(
"var self_ = window.gbar;\n" +
"self_.qs = function() {};");
}
public void testNoRemoveWindowPropertyAlias2() {
testSame(
"var self_ = window;\n" +
"self_.qs = function() {};");
}
public void testNoRemoveWindowPropertyAlias3() {
testSame(
"var self_ = window;\n" +
"self_['qs'] = function() {};");
}
public void testNoRemoveWindowPropertyAlias4() {
// TODO(johnlenz): fix this. "self_" should remain.
test(
"var self_ = window['gbar'] || {};\n" +
"self_.qs = function() {};",
"");
}
public void testNoRemoveWindowPropertyAlias4a() {
// TODO(johnlenz): fix this. "self_" should remain.
test(
"var self_; self_ = window.gbar || {};\n" +
"self_.qs = function() {};",
"");
}
public void testNoRemoveWindowPropertyAlias5() {
// TODO(johnlenz): fix this. "self_" should remain.
test(
"var self_ = window || {};\n" +
"self_['qs'] = function() {};",
"");
}
public void testNoRemoveWindowPropertyAlias5a() {
// TODO(johnlenz): fix this.
test(
"var self_; self_ = window || {};\n" +
"self_['qs'] = function() {};",
"");
}
public void testNoRemoveWindowPropertyAlias6() {
testSame(
"var self_ = (window.gbar = window.gbar || {});\n" +
"self_.qs = function() {};");
}
public void testNoRemoveWindowPropertyAlias6a() {
testSame(
"var self_; self_ = (window.gbar = window.gbar || {});\n" +
"self_.qs = function() {};");
}
public void testNoRemoveWindowPropertyAlias7() {
testSame(
"var self_ = (window = window || {});\n" +
"self_['qs'] = function() {};");
}
public void testNoRemoveWindowPropertyAlias7a() {
testSame(
"var self_; self_ = (window = window || {});\n" +
"self_['qs'] = function() {};");
}
public void testNoRemoveAlias0() {
testSame(
"var x = {}; function f() { return x; }; " +
"f().style.display = 'block';" +
"alert(x.style)");
}
public void testNoRemoveAlias1() {
testSame(
"var x = {}; function f() { return x; };" +
"var map = f();\n" +
"map.style.display = 'block';" +
"alert(x.style)");
}
public void testNoRemoveAlias2() {
testSame(
"var x = {};" +
"var map = (function () { return x; })();\n" +
"map.style = 'block';" +
"alert(x.style)");
}
public void testNoRemoveAlias3() {
testSame(
"var x = {}; function f() { return x; };" +
"var map = {}\n" +
"map[1] = f();\n" +
"map[1].style.display = 'block';");
}
public void testNoRemoveAliasOfExternal0() {
testSame(
"document.getElementById('foo').style.display = 'block';");
}
public void testNoRemoveAliasOfExternal1() {
testSame(
"var map = document.getElementById('foo');\n" +
"map.style.display = 'block';");
}
public void testNoRemoveAliasOfExternal2() {
testSame(
"var map = {}\n" +
"map[1] = document.getElementById('foo');\n" +
"map[1].style.display = 'block';");
}
public void testNoRemoveThrowReference1() {
testSame(
"var e = {}\n" +
"throw e;");
}
public void testNoRemoveThrowReference2() {
testSame(
"function e() {}\n" +
"throw new e();");
}
public void testClassDefinedInObjectLit1() {
test(
"var data = {Foo: function() {}};" +
"data.Foo.prototype.toString = function() {};",
"");
}
public void testClassDefinedInObjectLit2() {
test(
"var data = {}; data.bar = {Foo: function() {}};" +
"data.bar.Foo.prototype.toString = function() {};",
"");
}
public void testClassDefinedInObjectLit3() {
test(
"var data = {bar: {Foo: function() {}}};" +
"data.bar.Foo.prototype.toString = function() {};",
"");
}
public void testClassDefinedInObjectLit4() {
test(
"var data = {};" +
"data.baz = {bar: {Foo: function() {}}};" +
"data.baz.bar.Foo.prototype.toString = function() {};",
"");
}
public void testVarReferencedInClassDefinedInObjectLit1() {
testSame(
"var ref = 3;" +
"var data = {Foo: function() { this.x = ref; }};" +
"window.Foo = data.Foo;");
}
public void testVarReferencedInClassDefinedInObjectLit2() {
testSame(
"var ref = 3;" +
"var data = {Foo: function() { this.x = ref; }," +
" Bar: function() {}};" +
"window.Bar = data.Bar;");
}
public void testArrayExt() {
testSame(
"Array.prototype.foo = function() { return 1 };" +
"var y = [];" +
"switch (y.foo()) {" +
"}");
}
public void testArrayAliasExt() {
testSame(
"Array$X = Array;" +
"Array$X.prototype.foo = function() { return 1 };" +
"function Array$X() {}" +
"var y = [];" +
"switch (y.foo()) {" +
"}");
}
public void testExternalAliasInstanceof1() {
test(
"Array$X = Array;" +
"function Array$X() {}" +
"var y = [];" +
"if (y instanceof Array) {}",
"var y = [];" +
"if (y instanceof Array) {}"
);
}
public void testExternalAliasInstanceof2() {
testSame(
"Array$X = Array;" +
"function Array$X() {}" +
"var y = [];" +
"if (y instanceof Array$X) {}");
}
public void testExternalAliasInstanceof3() {
testSame(
"var b = Array;" +
"var y = [];" +
"if (y instanceof b) {}");
}
public void testAliasInstanceof4() {
testSame(
"function Foo() {};" +
"var b = Foo;" +
"var y = new Foo();" +
"if (y instanceof b) {}");
}
public void testAliasInstanceof5() {
// TODO(johnlenz): fix this. "b" should remain.
test(
"function Foo() {}" +
"function Bar() {}" +
"var b = x ? Foo : Bar;" +
"var y = new Foo();" +
"if (y instanceof b) {}",
"function Foo() {}" +
"var y = new Foo;" +
"if (false){}");
}
// We cannot leave x.a.prototype there because it will
// fail sanity var check.
public void testBrokenNamespaceWithPrototypeAssignment() {
test("var x = {}; x.a.prototype = 1", "");
}
public void testRemovePrototypeAliases() {
test(
"function g() {}" +
"function F() {} F.prototype.bar = g;" +
"window.g = g;",
"function g() {}" +
"window.g = g;");
}
public void testIssue284() {
test(
"var goog = {};" +
"goog.inherits = function(x, y) {};" +
"var ns = {};" +
"/** @constructor */" +
"ns.PageSelectionModel = function() {};" +
"/** @constructor */" +
"ns.PageSelectionModel.FooEvent = function() {};" +
"/** @constructor */" +
"ns.PageSelectionModel.SelectEvent = function() {};" +
"goog.inherits(ns.PageSelectionModel.ChangeEvent," +
" ns.PageSelectionModel.FooEvent);",
"");
}
public void testIssue838a() {
testSame("var z = window['z'] || (window['z'] = {});\n" +
"z['hello'] = 'Hello';\n" +
"z['world'] = 'World';");
}
public void testIssue838b() {
testSame(
"var z;" +
"window['z'] = z || (z = {});\n" +
"z['hello'] = 'Hello';\n" +
"z['world'] = 'World';");
}
public void testIssue874a() {
testSame(
"var a = a || {};\n" +
"var b = a;\n" +
"b.View = b.View || {}\n" +
"var c = b.View;\n" +
"c.Editor = function f(d, e) {\n" +
" return d + e\n" +
"};\n" +
"window.ImageEditor.View.Editor = a.View.Editor;");
}
public void testIssue874b() {
testSame(
"var b;\n" +
"var c = b = {};\n" +
"c.Editor = function f(d, e) {\n" +
" return d + e\n" +
"};\n" +
"window['Editor'] = b.Editor;");
}
public void testIssue874c() {
testSame(
"var b, c;\n" +
"c = b = {};\n" +
"c.Editor = function f(d, e) {\n" +
" return d + e\n" +
"};\n" +
"window['Editor'] = b.Editor;");
}
public void testIssue874d() {
testSame(
"var b = {}, c;\n" +
"c = b;\n" +
"c.Editor = function f(d, e) {\n" +
" return d + e\n" +
"};\n" +
"window['Editor'] = b.Editor;");
}
public void testIssue874e() {
testSame(
"var a;\n" +
"var b = a || (a = {});\n" +
"var c = b.View || (b.View = {});\n" +
"c.Editor = function f(d, e) {\n" +
" return d + e\n" +
"};\n" +
"window.ImageEditor.View.Editor = a.View.Editor;");
}
public void testBug6575051() {
testSame(
"var hackhack = window['__o_o_o__'] = window['__o_o_o__'] || {};\n" +
"window['__o_o_o__']['va'] = 1;\n" +
"hackhack['Vb'] = 1;");
}
@Override
protected CompilerPass getProcessor(Compiler compiler) {
return new MarkNoSideEffectCallsAndNameAnalyzerRunner(compiler);
}
private class MarkNoSideEffectCallsAndNameAnalyzerRunner
implements CompilerPass {
MarkNoSideEffectCalls markNoSideEffectCalls;
NameAnalyzer analyzer;
MarkNoSideEffectCallsAndNameAnalyzerRunner(Compiler compiler) {
this.markNoSideEffectCalls = new MarkNoSideEffectCalls(compiler);
this.analyzer = new NameAnalyzer(compiler, true);
}
@Override
public void process(Node externs, Node root) {
markNoSideEffectCalls.process(externs, root);
analyzer.process(externs, root);
}
}
} | // You are a professional Java test case writer, please create a test case named `testAssignWithCall` for the issue `Closure-1085`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-1085
//
// ## Issue-Title:
// Crash on the web closure compiler
//
// ## Issue-Description:
// With the web application (http://closure-compiler.appspot.com/home)
//
// Config:
//
// // ==ClosureCompiler==
// // @output\_file\_name default.js
// // @compilation\_level ADVANCED\_OPTIMIZATIONS
// // ==/ClosureCompiler==
//
//
// Code:
//
// var g=function(m){return m\*Math.random()|0},d=document,h=d.getElementById('h'),c=d.getElementById('c'),l;
// (l=function(){requestAnimationFrame(l);h.style.textShadow="0 0 1px #000,"+(g(10)-5)+"px "+(g(10)-5)+"px 0 #888,0 0 180px rgb("+g(255)+","+g(255)+","+g(255)+")"})();
// d.addEventListener('mousemove',function(v){c.style.marginTop=(v.pageY/10+15|0)+'px'})
//
//
// Cause:
//
// var l; // Déclare l variable
//
// // Store function in l var and call
// (l = function(){ ... }) ();
//
//
// Crash repport: (long)
//
// 23: java.lang.RuntimeException: INTERNAL COMPILER ERROR.
// Please report this problem.
// Unexpected variable l
// Node(NAME l): Input\_0:2:36
// (l=function(){requestAnimationFrame(l);h.style.textShadow="0 0 1px #000,"+(g(10)-5)+"px "+(g(10)-5)+"px 0 #888,0 0 180px rgb("+g(255)+","+g(255)+","+g(255)+")"})();
// Parent(CALL): Input\_0:2:14
// (l=function(){requestAnimationFrame(l);h.style.textShadow="0 0 1px #000,"+(g(10)-5)+"px "+(g(10)-5)+"px 0 #888,0 0 180px rgb("+g(255)+","+g(255)+","+g(255)+")"})();
//
// at com.google.javascript.jscomp.VarCheck.visit(VarCheck.java:159)
// at com.google.javascript.jscomp.NodeTraversal.traverseBranch(NodeTraversal.java:544)
// at com.google.javascript.jscomp.NodeTraversal.traverseBranch(NodeTraversal.java:538)
// at com.google.javascript.jscomp.NodeTraversal.traverseBranch(NodeTraversal.java:538)
// at com.google.javascript.jscomp.NodeTraversal.traverseBranch(NodeTraversal.java:538)
// at com.google.javascript.jscomp.NodeTraversal.traverseBranch(NodeTraversal.java:538)
// at com.google.javascript.jscomp.NodeTraversal.traverseRoots(NodeTraversal.java:318)
// at com.google.javascript.jscomp.NodeTraversal.traverseRoots(NodeTraversal.java:507)
// at com.google.javascript.jscomp.VarCheck.process(VarCheck.java:102)
// at com.google.javascript.jscomp.PhaseOptimizer$NamedPass.process(PhaseOptimizer.java:271)
// at com.google.javascript.jscomp.PhaseOptimizer.process(PhaseOptimizer.java:215)
// at com.google.javascript.jscomp.Compiler.optimize(Compiler.java:1918)
// at com.google.javascript.jscomp.Compiler.compileInternal(Compiler.java:751)
// at com.google.javascript.jscomp.Compiler.access$000(Compiler.java:85)
// at com.google.javascript.jscomp.Compiler$2.call(Compiler.java:652)
// at com.google.javascript.jscomp.Compiler$2.call(Compiler.java:649)
// at com.google.javascript.js
public void testAssignWithCall() {
| 1,237 | 114 | 1,234 | test/com/google/javascript/jscomp/NameAnalyzerTest.java | test | ```markdown
## Issue-ID: Closure-1085
## Issue-Title:
Crash on the web closure compiler
## Issue-Description:
With the web application (http://closure-compiler.appspot.com/home)
Config:
// ==ClosureCompiler==
// @output\_file\_name default.js
// @compilation\_level ADVANCED\_OPTIMIZATIONS
// ==/ClosureCompiler==
Code:
var g=function(m){return m\*Math.random()|0},d=document,h=d.getElementById('h'),c=d.getElementById('c'),l;
(l=function(){requestAnimationFrame(l);h.style.textShadow="0 0 1px #000,"+(g(10)-5)+"px "+(g(10)-5)+"px 0 #888,0 0 180px rgb("+g(255)+","+g(255)+","+g(255)+")"})();
d.addEventListener('mousemove',function(v){c.style.marginTop=(v.pageY/10+15|0)+'px'})
Cause:
var l; // Déclare l variable
// Store function in l var and call
(l = function(){ ... }) ();
Crash repport: (long)
23: java.lang.RuntimeException: INTERNAL COMPILER ERROR.
Please report this problem.
Unexpected variable l
Node(NAME l): Input\_0:2:36
(l=function(){requestAnimationFrame(l);h.style.textShadow="0 0 1px #000,"+(g(10)-5)+"px "+(g(10)-5)+"px 0 #888,0 0 180px rgb("+g(255)+","+g(255)+","+g(255)+")"})();
Parent(CALL): Input\_0:2:14
(l=function(){requestAnimationFrame(l);h.style.textShadow="0 0 1px #000,"+(g(10)-5)+"px "+(g(10)-5)+"px 0 #888,0 0 180px rgb("+g(255)+","+g(255)+","+g(255)+")"})();
at com.google.javascript.jscomp.VarCheck.visit(VarCheck.java:159)
at com.google.javascript.jscomp.NodeTraversal.traverseBranch(NodeTraversal.java:544)
at com.google.javascript.jscomp.NodeTraversal.traverseBranch(NodeTraversal.java:538)
at com.google.javascript.jscomp.NodeTraversal.traverseBranch(NodeTraversal.java:538)
at com.google.javascript.jscomp.NodeTraversal.traverseBranch(NodeTraversal.java:538)
at com.google.javascript.jscomp.NodeTraversal.traverseBranch(NodeTraversal.java:538)
at com.google.javascript.jscomp.NodeTraversal.traverseRoots(NodeTraversal.java:318)
at com.google.javascript.jscomp.NodeTraversal.traverseRoots(NodeTraversal.java:507)
at com.google.javascript.jscomp.VarCheck.process(VarCheck.java:102)
at com.google.javascript.jscomp.PhaseOptimizer$NamedPass.process(PhaseOptimizer.java:271)
at com.google.javascript.jscomp.PhaseOptimizer.process(PhaseOptimizer.java:215)
at com.google.javascript.jscomp.Compiler.optimize(Compiler.java:1918)
at com.google.javascript.jscomp.Compiler.compileInternal(Compiler.java:751)
at com.google.javascript.jscomp.Compiler.access$000(Compiler.java:85)
at com.google.javascript.jscomp.Compiler$2.call(Compiler.java:652)
at com.google.javascript.jscomp.Compiler$2.call(Compiler.java:649)
at com.google.javascript.js
```
You are a professional Java test case writer, please create a test case named `testAssignWithCall` for the issue `Closure-1085`, utilizing the provided issue report information and the following function signature.
```java
public void testAssignWithCall() {
```
| 1,234 | [
"com.google.javascript.jscomp.NameAnalyzer"
] | 9a1c6cfcb42b3cfb50e10d3996590305ff3a955f2537a733d2f9a1366224135f | public void testAssignWithCall() | // You are a professional Java test case writer, please create a test case named `testAssignWithCall` for the issue `Closure-1085`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-1085
//
// ## Issue-Title:
// Crash on the web closure compiler
//
// ## Issue-Description:
// With the web application (http://closure-compiler.appspot.com/home)
//
// Config:
//
// // ==ClosureCompiler==
// // @output\_file\_name default.js
// // @compilation\_level ADVANCED\_OPTIMIZATIONS
// // ==/ClosureCompiler==
//
//
// Code:
//
// var g=function(m){return m\*Math.random()|0},d=document,h=d.getElementById('h'),c=d.getElementById('c'),l;
// (l=function(){requestAnimationFrame(l);h.style.textShadow="0 0 1px #000,"+(g(10)-5)+"px "+(g(10)-5)+"px 0 #888,0 0 180px rgb("+g(255)+","+g(255)+","+g(255)+")"})();
// d.addEventListener('mousemove',function(v){c.style.marginTop=(v.pageY/10+15|0)+'px'})
//
//
// Cause:
//
// var l; // Déclare l variable
//
// // Store function in l var and call
// (l = function(){ ... }) ();
//
//
// Crash repport: (long)
//
// 23: java.lang.RuntimeException: INTERNAL COMPILER ERROR.
// Please report this problem.
// Unexpected variable l
// Node(NAME l): Input\_0:2:36
// (l=function(){requestAnimationFrame(l);h.style.textShadow="0 0 1px #000,"+(g(10)-5)+"px "+(g(10)-5)+"px 0 #888,0 0 180px rgb("+g(255)+","+g(255)+","+g(255)+")"})();
// Parent(CALL): Input\_0:2:14
// (l=function(){requestAnimationFrame(l);h.style.textShadow="0 0 1px #000,"+(g(10)-5)+"px "+(g(10)-5)+"px 0 #888,0 0 180px rgb("+g(255)+","+g(255)+","+g(255)+")"})();
//
// at com.google.javascript.jscomp.VarCheck.visit(VarCheck.java:159)
// at com.google.javascript.jscomp.NodeTraversal.traverseBranch(NodeTraversal.java:544)
// at com.google.javascript.jscomp.NodeTraversal.traverseBranch(NodeTraversal.java:538)
// at com.google.javascript.jscomp.NodeTraversal.traverseBranch(NodeTraversal.java:538)
// at com.google.javascript.jscomp.NodeTraversal.traverseBranch(NodeTraversal.java:538)
// at com.google.javascript.jscomp.NodeTraversal.traverseBranch(NodeTraversal.java:538)
// at com.google.javascript.jscomp.NodeTraversal.traverseRoots(NodeTraversal.java:318)
// at com.google.javascript.jscomp.NodeTraversal.traverseRoots(NodeTraversal.java:507)
// at com.google.javascript.jscomp.VarCheck.process(VarCheck.java:102)
// at com.google.javascript.jscomp.PhaseOptimizer$NamedPass.process(PhaseOptimizer.java:271)
// at com.google.javascript.jscomp.PhaseOptimizer.process(PhaseOptimizer.java:215)
// at com.google.javascript.jscomp.Compiler.optimize(Compiler.java:1918)
// at com.google.javascript.jscomp.Compiler.compileInternal(Compiler.java:751)
// at com.google.javascript.jscomp.Compiler.access$000(Compiler.java:85)
// at com.google.javascript.jscomp.Compiler$2.call(Compiler.java:652)
// at com.google.javascript.jscomp.Compiler$2.call(Compiler.java:649)
// at com.google.javascript.js
| Closure | /*
* Copyright 2006 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import com.google.javascript.rhino.Node;
/**
* Tests for {@link NameAnalyzer}
*
*/
public class NameAnalyzerTest extends CompilerTestCase {
private static String kExterns =
"var window, top;" +
"var document;" +
"var Function;" +
"var Array;" +
"var externfoo; methods.externfoo;";
public NameAnalyzerTest() {
super(kExterns);
}
@Override
protected void setUp() {
super.enableNormalize();
super.enableLineNumberCheck(true);
}
@Override
protected int getNumRepetitions() {
// pass reaches steady state after 1 iteration.
return 1;
}
public void testRemoveVarDeclaration1() {
test("var foo = 3;", "");
}
public void testRemoveVarDeclaration2() {
test("var foo = 3, bar = 4; externfoo = foo;",
"var foo = 3; externfoo = foo;");
}
public void testRemoveVarDeclaration3() {
test("var a = f(), b = 1, c = 2; b; c", "f();var b = 1, c = 2; b; c");
}
public void testRemoveVarDeclaration4() {
test("var a = 0, b = f(), c = 2; a; c", "var a = 0;f();var c = 2; a; c");
}
public void testRemoveVarDeclaration5() {
test("var a = 0, b = 1, c = f(); a; b", "var a = 0, b = 1; f(); a; b");
}
public void testRemoveVarDeclaration6() {
test("var a = 0, b = a = 1; a", "var a = 0; a = 1; a");
}
public void testRemoveVarDeclaration7() {
test("var a = 0, b = a = 1", "");
}
public void testRemoveVarDeclaration8() {
test("var a;var b = 0, c = a = b = 1", "");
}
public void testRemoveDeclaration1() {
test("var a;var b = 0, c = a = b = 1", "");
}
public void testRemoveDeclaration2() {
test("var a,b,c; c = a = b = 1", "");
}
public void testRemoveDeclaration3() {
test("var a,b,c; c = a = b = {}; a.x = 1;", "");
}
public void testRemoveDeclaration4() {
testSame("var a,b,c; c = a = b = {}; a.x = 1;alert(c.x);");
}
public void testRemoveDeclaration5() {
test("var a,b,c; c = a = b = null; use(b)", "var b;b=null;use(b)");
}
public void testRemoveDeclaration6() {
test("var a,b,c; c = a = b = 'str';use(b)", "var b;b='str';use(b)");
}
public void testRemoveDeclaration7() {
test("var a,b,c; c = a = b = true;use(b)", "var b;b=true;use(b)");
}
public void testRemoveFunction1() {
test("var foo = function(){};", "");
}
public void testRemoveFunction2() {
test("var foo; foo = function(){};", "");
}
public void testRemoveFunction3() {
test("var foo = {}; foo.bar = function() {};", "");
}
public void testRemoveFunction4() {
test("var a = {}; a.b = {}; a.b.c = function() {};", "");
}
public void testReferredToByWindow() {
testSame("var foo = {}; foo.bar = function() {}; window['fooz'] = foo.bar");
}
public void testExtern() {
testSame("externfoo = 5");
}
public void testRemoveNamedFunction() {
test("function foo(){}", "");
}
public void testRemoveRecursiveFunction1() {
test("function f(){f()}", "");
}
public void testRemoveRecursiveFunction2() {
test("var f = function (){f()}", "");
}
public void testRemoveRecursiveFunction2a() {
test("var f = function g(){g()}", "");
}
public void testRemoveRecursiveFunction3() {
test("var f;f = function (){f()}", "");
}
public void testRemoveRecursiveFunction4() {
// don't removed if name definition doesn't exist.
testSame("f = function (){f()}");
}
public void testRemoveRecursiveFunction5() {
test("function g(){f()}function f(){g()}", "");
}
public void testRemoveRecursiveFunction6() {
test("var f=function(){g()};function g(){f()}", "");
}
public void testRemoveRecursiveFunction7() {
test("var g = function(){f()};var f = function(){g()}", "");
}
public void testRemoveRecursiveFunction8() {
test("var o = {};o.f = function(){o.f()}", "");
}
public void testRemoveRecursiveFunction9() {
testSame("var o = {};o.f = function(){o.f()};o.f()");
}
public void testSideEffectClassification1() {
test("foo();", "foo();");
}
public void testSideEffectClassification2() {
test("var a = foo();", "foo();");
}
public void testSideEffectClassification3() {
testSame("var a = foo();window['b']=a;");
}
public void testSideEffectClassification4() {
testSame("function sef(){} sef();");
}
public void testSideEffectClassification5() {
testSame("function nsef(){} var a = nsef();window['b']=a;");
}
public void testSideEffectClassification6() {
test("function sef(){} sef();", "function sef(){} sef();");
}
public void testSideEffectClassification7() {
testSame("function sef(){} var a = sef();window['b']=a;");
}
public void testNoSideEffectAnnotation1() {
test("function f(){} var a = f();",
"function f(){} f()");
}
public void testNoSideEffectAnnotation2() {
test("/**@nosideeffects*/function f(){}", "var a = f();",
"", null, null);
}
public void testNoSideEffectAnnotation3() {
test("var f = function(){}; var a = f();",
"var f = function(){}; f();");
}
public void testNoSideEffectAnnotation4() {
test("var f = /**@nosideeffects*/function(){};", "var a = f();",
"", null, null);
}
public void testNoSideEffectAnnotation5() {
test("var f; f = function(){}; var a = f();",
"var f; f = function(){}; f();");
}
public void testNoSideEffectAnnotation6() {
test("var f; f = /**@nosideeffects*/function(){};", "var a = f();",
"", null, null);
}
public void testNoSideEffectAnnotation7() {
test("var f;" +
"f = /**@nosideeffects*/function(){};",
"f = function(){};" +
"var a = f();",
"f = function(){}; f();", null, null);
}
public void testNoSideEffectAnnotation8() {
test("var f;" +
"f = function(){};" +
"f = /**@nosideeffects*/function(){};",
"var a = f();",
"f();", null, null);
}
public void testNoSideEffectAnnotation9() {
test("var f;" +
"f = /**@nosideeffects*/function(){};" +
"f = /**@nosideeffects*/function(){};",
"var a = f();",
"", null, null);
test("var f; f = /**@nosideeffects*/function(){};", "var a = f();",
"", null, null);
}
public void testNoSideEffectAnnotation10() {
test("var o = {}; o.f = function(){}; var a = o.f();",
"var o = {}; o.f = function(){}; o.f();");
}
public void testNoSideEffectAnnotation11() {
test("var o = {}; o.f = /**@nosideeffects*/function(){};",
"var a = o.f();", "", null, null);
}
public void testNoSideEffectAnnotation12() {
test("function c(){} var a = new c",
"function c(){} new c");
}
public void testNoSideEffectAnnotation13() {
test("/**@nosideeffects*/function c(){}", "var a = new c",
"", null, null);
}
public void testNoSideEffectAnnotation14() {
String common = "function c(){};" +
"c.prototype.f = /**@nosideeffects*/function(){};";
test(common, "var o = new c; var a = o.f()", "new c", null, null);
}
public void testNoSideEffectAnnotation15() {
test("function c(){}; c.prototype.f = function(){}; var a = (new c).f()",
"function c(){}; c.prototype.f = function(){}; (new c).f()");
}
public void testNoSideEffectAnnotation16() {
test("/**@nosideeffects*/function c(){}" +
"c.prototype.f = /**@nosideeffects*/function(){};",
"var a = (new c).f()",
"",
null, null);
}
public void testFunctionPrototype() {
testSame("var a = 5; Function.prototype.foo = function() {return a;}");
}
public void testTopLevelClass1() {
test("var Point = function() {}; Point.prototype.foo = function() {}", "");
}
public void testTopLevelClass2() {
testSame("var Point = {}; Point.prototype.foo = function() {};" +
"externfoo = new Point()");
}
public void testTopLevelClass3() {
test("function Point() {this.me_ = Point}", "");
}
public void testTopLevelClass4() {
test("function f(){} function A(){} A.prototype = {x: function() {}}; f();",
"function f(){} f();");
}
public void testTopLevelClass5() {
testSame("function f(){} function A(){}" +
"A.prototype = {x: function() { f(); }}; new A();");
}
public void testTopLevelClass6() {
testSame("function f(){} function A(){}" +
"A.prototype = {x: function() { f(); }}; new A().x();");
}
public void testTopLevelClass7() {
test("A.prototype.foo = function(){}; function A() {}", "");
}
public void testNamespacedClass1() {
test("var foo = {};foo.bar = {};foo.bar.prototype.baz = {}", "");
}
public void testNamespacedClass2() {
testSame("var foo = {};foo.bar = {};foo.bar.prototype.baz = {};" +
"window.z = new foo.bar()");
}
public void testNamespacedClass3() {
test("var a = {}; a.b = function() {}; a.b.prototype = {x: function() {}};",
"");
}
public void testNamespacedClass4() {
testSame("function f(){} var a = {}; a.b = function() {};" +
"a.b.prototype = {x: function() { f(); }}; new a.b();");
}
public void testNamespacedClass5() {
testSame("function f(){} var a = {}; a.b = function() {};" +
"a.b.prototype = {x: function() { f(); }}; new a.b().x();");
}
public void testAssignmentToThisPrototype() {
testSame("Function.prototype.inherits = function(parentCtor) {" +
" function tempCtor() {};" +
" tempCtor.prototype = parentCtor.prototype;" +
" this.superClass_ = parentCtor.prototype;" +
" this.prototype = new tempCtor();" +
" this.prototype.constructor = this;" +
"};");
}
public void testAssignmentToCallResultPrototype() {
testSame("function f() { return function(){}; } f().prototype = {};");
}
public void testAssignmentToExternPrototype() {
testSame("externfoo.prototype = {};");
}
public void testAssignmentToUnknownPrototype() {
testSame(
"/** @suppress {duplicate} */ var window;" +
"window['a'].prototype = {};");
}
public void testBug2099540() {
testSame(
"/** @suppress {duplicate} */ var document;\n" +
"/** @suppress {duplicate} */ var window;\n" +
"var klass;\n" +
"window[klass].prototype = " +
"document.createElement(tagName)['__proto__'];");
}
public void testOtherGlobal() {
testSame("goog.global.foo = bar(); function bar(){}");
}
public void testExternName1() {
testSame("top.z = bar(); function bar(){}");
}
public void testExternName2() {
testSame("top['z'] = bar(); function bar(){}");
}
public void testInherits1() {
test("var a = {}; var b = {}; b.inherits(a)", "");
}
public void testInherits2() {
test("var a = {}; var b = {}; var goog = {}; goog.inherits(b, a)", "");
}
public void testInherits3() {
testSame("var a = {}; this.b = {}; b.inherits(a);");
}
public void testInherits4() {
testSame("var a = {}; this.b = {}; var goog = {}; goog.inherits(b, a);");
}
public void testInherits5() {
test("this.a = {}; var b = {}; b.inherits(a);",
"this.a = {}");
}
public void testInherits6() {
test("this.a = {}; var b = {}; var goog = {}; goog.inherits(b, a);",
"this.a = {}");
}
public void testInherits7() {
testSame("var a = {}; this.b = {}; var goog = {};" +
" goog.inherits = function() {}; goog.inherits(b, a);");
}
public void testInherits8() {
// Make sure that exceptions aren't thrown if inherits() is used as
// an R-value
test("this.a = {}; var b = {}; var c = b.inherits(a);", "this.a = {};");
}
public void testMixin1() {
testSame("var goog = {}; goog.mixin = function() {};" +
"Function.prototype.mixin = function(base) {" +
" goog.mixin(this.prototype, base); " +
"};");
}
public void testMixin2() {
testSame("var a = {}; this.b = {}; var goog = {};" +
" goog.mixin = function() {}; goog.mixin(b.prototype, a.prototype);");
}
public void testMixin3() {
test("this.a = {}; var b = {}; var goog = {};" +
" goog.mixin = function() {}; goog.mixin(b.prototype, a.prototype);",
"this.a = {};");
}
public void testMixin4() {
testSame("this.a = {}; var b = {}; var goog = {};" +
"goog.mixin = function() {};" +
"goog.mixin(b.prototype, a.prototype);" +
"new b()");
}
public void testMixin5() {
test("this.a = {}; var b = {}; var c = {}; var goog = {};" +
"goog.mixin = function() {};" +
"goog.mixin(b.prototype, a.prototype);" +
"goog.mixin(c.prototype, a.prototype);" +
"new b()",
"this.a = {}; var b = {}; var goog = {};" +
"goog.mixin = function() {};" +
"goog.mixin(b.prototype, a.prototype);" +
"new b()");
}
public void testMixin6() {
testSame("this.a = {}; var b = {}; var c = {}; var goog = {};" +
"goog.mixin = function() {};" +
"goog.mixin(c.prototype, a.prototype) + " +
"goog.mixin(b.prototype, a.prototype);" +
"new b()");
}
public void testMixin7() {
test("this.a = {}; var b = {}; var c = {}; var goog = {};" +
"goog.mixin = function() {};" +
"var d = goog.mixin(c.prototype, a.prototype) + " +
"goog.mixin(b.prototype, a.prototype);" +
"new b()",
"this.a = {}; var b = {}; var goog = {};" +
"goog.mixin = function() {};" +
"goog.mixin(b.prototype, a.prototype);" +
"new b()");
}
public void testConstants1() {
testSame("var bar = function(){}; var EXP_FOO = true; if (EXP_FOO) bar();");
}
public void testConstants2() {
test("var bar = function(){}; var EXP_FOO = true; var EXP_BAR = true;" +
"if (EXP_FOO) bar();",
"var bar = function(){}; var EXP_FOO = true; if (EXP_FOO) bar();");
}
public void testExpressions1() {
test("var foo={}; foo.A='A'; foo.AB=foo.A+'B'; foo.ABC=foo.AB+'C'",
"");
}
public void testExpressions2() {
testSame("var foo={}; foo.A='A'; foo.AB=foo.A+'B'; this.ABC=foo.AB+'C'");
}
public void testExpressions3() {
testSame("var foo = 2; window.bar(foo + 3)");
}
public void testSetCreatingReference() {
testSame("var foo; var bar = function(){foo=6;}; bar();");
}
public void testAnonymous1() {
testSame("function foo() {}; function bar() {}; foo(function() {bar()})");
}
public void testAnonymous2() {
test("var foo;(function(){foo=6;})()", "(function(){})()");
}
public void testAnonymous3() {
testSame("var foo; (function(){ if(!foo)foo=6; })()");
}
public void testAnonymous4() {
testSame("var foo; (function(){ foo=6; })(); externfoo=foo;");
}
public void testAnonymous5() {
testSame("var foo;" +
"(function(){ foo=function(){ bar() }; function bar(){} })();" +
"foo();");
}
public void testAnonymous6() {
testSame("function foo(){}" +
"function bar(){}" +
"foo(function(){externfoo = bar});");
}
public void testAnonymous7() {
testSame("var foo;" +
"(function (){ function bar(){ externfoo = foo; } bar(); })();");
}
public void testAnonymous8() {
testSame("var foo;" +
"(function (){ var g=function(){ externfoo = foo; }; g(); })();");
}
public void testAnonymous9() {
testSame("function foo(){}" +
"function bar(){}" +
"foo(function(){ function baz(){ externfoo = bar; } baz(); });");
}
public void testFunctions1() {
testSame("var foo = null; function baz() {}" +
"function bar() {foo=baz();} bar();");
}
public void testFunctions2() {
testSame("var foo; foo = function() {var a = bar()};" +
"var bar = function(){}; foo();");
}
public void testGetElem1() {
testSame("var foo = {}; foo.bar = {}; foo.bar.baz = {a: 5, b: 10};" +
"var fn = function() {window[foo.bar.baz.a] = 5;}; fn()");
}
public void testGetElem2() {
testSame("var foo = {}; foo.bar = {}; foo.bar.baz = {a: 5, b: 10};" +
"var fn = function() {this[foo.bar.baz.a] = 5;}; fn()");
}
public void testGetElem3() {
testSame("var foo = {'i': 0, 'j': 1}; foo['k'] = 2; top.foo = foo;");
}
public void testIf1() {
test("var foo = {};if(e)foo.bar=function(){};", "if(e);");
}
public void testIf2() {
test("var e = false;var foo = {};if(e)foo.bar=function(){};",
"var e = false;if(e);");
}
public void testIf3() {
test("var e = false;var foo = {};if(e + 1)foo.bar=function(){};",
"var e = false;if(e + 1);");
}
public void testIf4() {
test("var e = false, f;var foo = {};if(f=e)foo.bar=function(){};",
"var e = false;if(e);");
}
public void testIf4a() {
// TODO(johnlenz): fix this.
testSame("var e = [], f;if(f=e);f[0] = 1;");
}
public void testIf4b() {
// TODO(johnlenz): fix this.
test("var e = [], f;if(e=f);f[0] = 1;",
"var f;if(f);f[0] = 1;");
}
public void testIf4c() {
test("var e = [], f;if(f=e);e[0] = 1;",
"var e = [];if(e);e[0] = 1;");
}
public void testIf5() {
test("var e = false, f;var foo = {};if(f = e + 1)foo.bar=function(){};",
"var e = false;if(e + 1);");
}
public void testIfElse() {
test("var foo = {};if(e)foo.bar=function(){};else foo.bar=function(){};",
"if(e);else;");
}
public void testWhile() {
test("var foo = {};while(e)foo.bar=function(){};", "while(e);");
}
public void testFor() {
test("var foo = {};for(e in x)foo.bar=function(){};", "for(e in x);");
}
public void testDo() {
test("var cond = false;do {var a = 1} while (cond)",
"var cond = false;do {} while (cond)");
}
public void testSetterInForStruct1() {
test("var j = 0; for (var i = 1; i = 0; j++);",
"var j = 0; for (; 0; j++);");
}
public void testSetterInForStruct2() {
test("var Class = function() {}; " +
"for (var i = 1; Class.prototype.property_ = 0; i++);",
"for (var i = 1; 0; i++);");
}
public void testSetterInForStruct3() {
test("var j = 0; for (var i = 1 + f() + g() + h(); i = 0; j++);",
"var j = 0; f(); g(); h(); for (; 0; j++);");
}
public void testSetterInForStruct4() {
test("var i = 0;var j = 0; for (i = 1 + f() + g() + h(); i = 0; j++);",
"var j = 0; f(); g(); h(); for (; 0; j++);");
}
public void testSetterInForStruct5() {
test("var i = 0, j = 0; for (i = f(), j = g(); 0;);",
"for (f(), g(); 0;);");
}
public void testSetterInForStruct6() {
test("var i = 0, j = 0, k = 0; for (i = f(), j = g(), k = h(); i = 0;);",
"for (f(), g(), h(); 0;);");
}
public void testSetterInForStruct7() {
test("var i = 0, j = 0, k = 0; for (i = 1, j = 2, k = 3; i = 0;);",
"for (1, 2, 3; 0;);");
}
public void testSetterInForStruct8() {
test("var i = 0, j = 0, k = 0; for (i = 1, j = i, k = 2; i = 0;);",
"var i = 0; for(i = 1, i , 2; i = 0;);");
}
public void testSetterInForStruct9() {
test("var Class = function() {}; " +
"for (var i = 1; Class.property_ = 0; i++);",
"for (var i = 1; 0; i++);");
}
public void testSetterInForStruct10() {
test("var Class = function() {}; " +
"for (var i = 1; Class.property_ = 0; i = 2);",
"for (; 0;);");
}
public void testSetterInForStruct11() {
test("var Class = function() {}; " +
"for (;Class.property_ = 0;);",
"for (;0;);");
}
public void testSetterInForStruct12() {
test("var a = 1; var Class = function() {}; " +
"for (;Class.property_ = a;);",
"var a = 1; for (; a;);");
}
public void testSetterInForStruct13() {
test("var a = 1; var Class = function() {}; " +
"for (Class.property_ = a; 0 ;);",
"for (; 0;);");
}
public void testSetterInForStruct14() {
test("var a = 1; var Class = function() {}; " +
"for (; 0; Class.property_ = a);",
"for (; 0;);");
}
public void testSetterInForStruct15() {
test("var Class = function() {}; " +
"for (var i = 1; 0; Class.prototype.property_ = 0);",
"for (; 0; 0);");
}
public void testSetterInForStruct16() {
test("var Class = function() {}; " +
"for (var i = 1; i = 0; Class.prototype.property_ = 0);",
"for (; 0; 0);");
}
public void testSetterInForIn1() {
test("var foo = {}; var bar; for(e in bar = foo.a);",
"var foo = {}; for(e in foo.a);");
}
public void testSetterInForIn2() {
testSame("var foo = {}; var bar; for(e in bar = foo.a); bar");
}
public void testSetterInForIn3() {
testSame("var foo = {}; var bar; for(e in bar = foo.a); bar.b = 3");
}
public void testSetterInForIn4() {
testSame("var foo = {}; var bar; for (e in bar = foo.a); bar.b = 3; foo.a");
}
public void testSetterInForIn5() {
// TODO(user) Fix issue similar to b/2316773: bar should be preserved
// but isn't due to missing references between e and foo.a
test("var foo = {}; var bar; for (e in foo.a) { bar = e } bar.b = 3; foo.a",
"var foo={};for(e in foo.a);foo.a");
}
public void testSetterInForIn6() {
testSame("var foo = {};for(e in foo);");
}
public void testSetterInIfPredicate() {
// TODO(user) Make NameAnalyzer smarter so it can remove "Class".
testSame("var a = 1;" +
"var Class = function() {}; " +
"if (Class.property_ = a);");
}
public void testSetterInWhilePredicate() {
test("var a = 1;" +
"var Class = function() {}; " +
"while (Class.property_ = a);",
"var a = 1; for (;a;) {}");
}
public void testSetterInDoWhilePredicate() {
// TODO(user) Make NameAnalyzer smarter so it can remove "Class".
testSame("var a = 1;" +
"var Class = function() {}; " +
"do {} while(Class.property_ = a);");
}
public void testSetterInSwitchInput() {
// TODO(user) Make NameAnalyzer smarter so it can remove "Class".
testSame("var a = 1;" +
"var Class = function() {}; " +
"switch (Class.property_ = a) {" +
" default:" +
"}");
}
public void testComplexAssigns() {
// Complex assigns are not removed by the current pass.
testSame("var x = 0; x += 3; x *= 5;");
}
public void testNestedAssigns1() {
test("var x = 0; var y = x = 3; window.alert(y);",
"var y = 3; window.alert(y);");
}
public void testNestedAssigns2() {
testSame("var x = 0; var y = x = {}; x.b = 3; window.alert(y);");
}
public void testComplexNestedAssigns1() {
// TODO(nicksantos): Make NameAnalyzer smarter, so that we can eliminate y.
testSame("var x = 0; var y = 2; y += x = 3; window.alert(x);");
}
public void testComplexNestedAssigns2() {
test("var x = 0; var y = 2; y += x = 3; window.alert(y);",
"var y = 2; y += 3; window.alert(y);");
}
public void testComplexNestedAssigns3() {
test("var x = 0; var y = x += 3; window.alert(x);",
"var x = 0; x += 3; window.alert(x);");
}
public void testComplexNestedAssigns4() {
testSame("var x = 0; var y = x += 3; window.alert(y);");
}
public void testUnintendedUseOfInheritsInLocalScope1() {
testSame("goog.mixin = function() {}; " +
"(function() { var x = {}; var y = {}; goog.mixin(x, y); })();");
}
public void testUnintendedUseOfInheritsInLocalScope2() {
testSame("goog.mixin = function() {}; " +
"var x = {}; var y = {}; (function() { goog.mixin(x, y); })();");
}
public void testUnintendedUseOfInheritsInLocalScope3() {
testSame("goog.mixin = function() {}; " +
"var x = {}; var y = {}; (function() { goog.mixin(x, y); })(); " +
"window.alert(x);");
}
public void testUnintendedUseOfInheritsInLocalScope4() {
// Ensures that the "goog$mixin" variable doesn't get stripped out,
// even when it's only used in a local scope.
testSame("var goog$mixin = function() {}; " +
"(function() { var x = {}; var y = {}; goog$mixin(x, y); })();");
}
public void testPrototypePropertySetInLocalScope1() {
testSame("(function() { var x = function(){}; x.prototype.bar = 3; })();");
}
public void testPrototypePropertySetInLocalScope2() {
testSame("var x = function(){}; (function() { x.prototype.bar = 3; })();");
}
public void testPrototypePropertySetInLocalScope3() {
test("var x = function(){ x.prototype.bar = 3; };", "");
}
public void testPrototypePropertySetInLocalScope4() {
test("var x = {}; x.foo = function(){ x.foo.prototype.bar = 3; };", "");
}
public void testPrototypePropertySetInLocalScope5() {
test("var x = {}; x.prototype.foo = 3;", "");
}
public void testPrototypePropertySetInLocalScope6() {
testSame("var x = {}; x.prototype.foo = 3; bar(x.prototype.foo)");
}
public void testPrototypePropertySetInLocalScope7() {
testSame("var x = {}; x.foo = 3; bar(x.foo)");
}
public void testRValueReference1() {
testSame("var a = 1; a");
}
public void testRValueReference2() {
testSame("var a = 1; 1+a");
}
public void testRValueReference3() {
testSame("var x = {}; x.prototype.foo = 3; var a = x.prototype.foo; 1+a");
}
public void testRValueReference4() {
testSame("var x = {}; x.prototype.foo = 3; x.prototype.foo");
}
public void testRValueReference5() {
testSame("var x = {}; x.prototype.foo = 3; 1+x.prototype.foo");
}
public void testRValueReference6() {
testSame("var x = {}; var idx = 2; x[idx]");
}
public void testUnhandledTopNode() {
testSame("function Foo() {}; Foo.prototype.isBar = function() {};" +
"function Bar() {}; Bar.prototype.isFoo = function() {};" +
"var foo = new Foo(); var bar = new Bar();" +
// The boolean AND here is currently unhandled by this pass, but
// it should not cause it to blow up.
"var cond = foo.isBar() && bar.isFoo();" +
"if (cond) {window.alert('hello');}");
}
public void testPropertyDefinedInGlobalScope() {
testSame("function Foo() {}; var x = new Foo(); x.cssClass = 'bar';" +
"window.alert(x);");
}
public void testConditionallyDefinedFunction1() {
testSame("var g; externfoo.x || (externfoo.x = function() { g; })");
}
public void testConditionallyDefinedFunction2() {
testSame("var g; 1 || (externfoo.x = function() { g; })");
}
public void testConditionallyDefinedFunction3() {
testSame("var a = {};" +
"rand() % 2 || (a.f = function() { externfoo = 1; } || alert());");
}
public void testGetElemOnThis() {
testSame("var a = 3; this['foo'] = a;");
testSame("this['foo'] = 3;");
}
public void testRemoveInstanceOfOnly() {
test("function Foo() {}; Foo.prototype.isBar = function() {};" +
"var x; if (x instanceof Foo) { window.alert(x); }",
";var x; if (false) { window.alert(x); }");
}
public void testRemoveLocalScopedInstanceOfOnly() {
test("function Foo() {}; function Bar(x) { this.z = x instanceof Foo; };" +
"externfoo.x = new Bar({});",
";function Bar(x) { this.z = false }; externfoo.x = new Bar({});");
}
public void testRemoveInstanceOfWithReferencedMethod() {
test("function Foo() {}; Foo.prototype.isBar = function() {};" +
"var x; if (x instanceof Foo) { window.alert(x.isBar()); }",
";var x; if (false) { window.alert(x.isBar()); }");
}
public void testDoNotChangeReferencedInstanceOf() {
testSame("function Foo() {}; Foo.prototype.isBar = function() {};" +
"var x = new Foo(); if (x instanceof Foo) { window.alert(x); }");
}
public void testDoNotChangeReferencedLocalScopedInstanceOf() {
testSame("function Foo() {}; externfoo.x = new Foo();" +
"function Bar() { if (x instanceof Foo) { window.alert(x); } };" +
"externfoo.y = new Bar();");
}
public void testDoNotChangeLocalScopeReferencedInstanceOf() {
testSame("function Foo() {}; Foo.prototype.isBar = function() {};" +
"function Bar() { this.z = new Foo(); }; externfoo.x = new Bar();" +
"if (x instanceof Foo) { window.alert(x); }");
}
public void testDoNotChangeLocalScopeReferencedLocalScopedInstanceOf() {
testSame("function Foo() {}; Foo.prototype.isBar = function() {};" +
"function Bar() { this.z = new Foo(); };" +
"Bar.prototype.func = function(x) {" +
"if (x instanceof Foo) { window.alert(x); }" +
"}; new Bar().func();");
}
public void testDoNotChangeLocalScopeReferencedLocalScopedInstanceOf2() {
test(
"function Foo() {}" +
"var createAxis = function(f) { return window.passThru(f); };" +
"var axis = createAxis(function(test) {" +
" return test instanceof Foo;" +
"});",
"var createAxis = function(f) { return window.passThru(f); };" +
"createAxis(function(test) {" +
" return false;" +
"});");
}
public void testDoNotChangeInstanceOfGetElem() {
testSame("var goog = {};" +
"function f(obj, name) {" +
" if (obj instanceof goog[name]) {" +
" return name;" +
" }" +
"}" +
"window['f'] = f;");
}
public void testWeirdnessOnLeftSideOfPrototype() {
// This checks a bug where 'x' was removed, but the function referencing
// it was not, causing problems.
testSame("var x = 3; " +
"(function() { this.bar = 3; }).z = function() {" +
" return x;" +
"};");
}
public void testShortCircuit1() {
test("var a = b() || 1", "b()");
}
public void testShortCircuit2() {
test("var a = 1 || c()", "1 || c()");
}
public void testShortCircuit3() {
test("var a = b() || c()", "b() || c()");
}
public void testShortCircuit4() {
test("var a = b() || 3 || c()", "b() || 3 || c()");
}
public void testShortCircuit5() {
test("var a = b() && 1", "b()");
}
public void testShortCircuit6() {
test("var a = 1 && c()", "1 && c()");
}
public void testShortCircuit7() {
test("var a = b() && c()", "b() && c()");
}
public void testShortCircuit8() {
test("var a = b() && 3 && c()", "b() && 3 && c()");
}
public void testRhsReference1() {
testSame("var a = 1; a");
}
public void testRhsReference2() {
testSame("var a = 1; a || b()");
}
public void testRhsReference3() {
testSame("var a = 1; 1 || a");
}
public void testRhsReference4() {
test("var a = 1; var b = a || foo()", "var a = 1; a || foo()");
}
public void testRhsReference5() {
test("var a = 1, b = 5; a; foo(b)", "var a = 1, b = 5; a; foo(b)");
}
public void testRhsAssign1() {
test("var foo, bar; foo || (bar = 1)",
"var foo; foo || 1");
}
public void testRhsAssign2() {
test("var foo, bar, baz; foo || (baz = bar = 1)",
"var foo; foo || 1");
}
public void testRhsAssign3() {
testSame("var foo = null; foo || (foo = 1)");
}
public void testRhsAssign4() {
test("var foo = null; foo = (foo || 1)", "");
}
public void testRhsAssign5() {
test("var a = 3, foo, bar; foo || (bar = a)", "var a = 3, foo; foo || a");
}
public void testRhsAssign6() {
test("function Foo(){} var foo = null;" +
"var f = function () {foo || (foo = new Foo()); return foo}",
"");
}
public void testRhsAssign7() {
testSame("function Foo(){} var foo = null;" +
"var f = function () {foo || (foo = new Foo())}; f()");
}
public void testRhsAssign8() {
testSame("function Foo(){} var foo = null;" +
"var f = function () {(foo = new Foo()) || g()}; f()");
}
public void testRhsAssign9() {
test("function Foo(){} var foo = null;" +
"var f = function () {1 + (foo = new Foo()); return foo}",
"");
}
public void testAssignWithOr1() {
testSame("var foo = null;" +
"var f = window.a || function () {return foo}; f()");
}
public void testAssignWithOr2() {
test("var foo = null;" +
"var f = window.a || function () {return foo};",
"var foo = null"); // why is this left?
}
public void testAssignWithAnd1() {
testSame("var foo = null;" +
"var f = window.a && function () {return foo}; f()");
}
public void testAssignWithAnd2() {
test("var foo = null;" +
"var f = window.a && function () {return foo};",
"var foo = null;"); // why is this left?
}
public void testAssignWithHook1() {
testSame("function Foo(){} var foo = null;" +
"var f = window.a ? " +
" function () {return new Foo()} : function () {return foo}; f()");
}
public void testAssignWithHook2() {
test("function Foo(){} var foo = null;" +
"var f = window.a ? " +
" function () {return new Foo()} : function () {return foo};",
"");
}
public void testAssignWithHook2a() {
test("function Foo(){} var foo = null;" +
"var f; f = window.a ? " +
" function () {return new Foo()} : function () {return foo};",
"");
}
public void testAssignWithHook3() {
testSame("function Foo(){} var foo = null; var f = {};" +
"f.b = window.a ? " +
" function () {return new Foo()} : function () {return foo}; f.b()");
}
public void testAssignWithHook4() {
test("function Foo(){} var foo = null; var f = {};" +
"f.b = window.a ? " +
" function () {return new Foo()} : function () {return foo};",
"");
}
public void testAssignWithHook5() {
testSame("function Foo(){} var foo = null; var f = {};" +
"f.b = window.a ? function () {return new Foo()} :" +
" window.b ? function () {return foo} :" +
" function() { return Foo }; f.b()");
}
public void testAssignWithHook6() {
test("function Foo(){} var foo = null; var f = {};" +
"f.b = window.a ? function () {return new Foo()} :" +
" window.b ? function () {return foo} :" +
" function() { return Foo };",
"");
}
public void testAssignWithHook7() {
testSame("function Foo(){} var foo = null;" +
"var f = window.a ? new Foo() : foo;" +
"f()");
}
public void testAssignWithHook8() {
test("function Foo(){} var foo = null;" +
"var f = window.a ? new Foo() : foo;",
"function Foo(){}" +
"window.a && new Foo()");
}
public void testAssignWithHook9() {
test("function Foo(){} var foo = null; var f = {};" +
"f.b = window.a ? new Foo() : foo;",
"function Foo(){} window.a && new Foo()");
}
public void testAssign1() {
test("function Foo(){} var foo = null; var f = {};" +
"f.b = window.a;",
"");
}
public void testAssign2() {
test("function Foo(){} var foo = null; var f = {};" +
"f.b = window;",
"");
}
public void testAssign3() {
test("var f = {};" +
"f.b = window;",
"");
}
public void testAssign4() {
test("function Foo(){} var foo = null; var f = {};" +
"f.b = new Foo();",
"function Foo(){} new Foo()");
}
public void testAssign5() {
test("function Foo(){} var foo = null; var f = {};" +
"f.b = foo;",
"");
}
public void testAssignWithCall() {
test("var fun, x; (fun = function(){ x; })();",
"var x; (function(){ x; })();");
}
// Currently this crashes the compiler because it erroneoursly removes var x
// and later a sanity check fails.
public void testAssignWithCall2() {
test("var fun, x; (123, fun = function(){ x; })();",
"(123, function(){ x; })();");
}
public void testNestedAssign1() {
test("var a, b = a = 1, c = 2", "");
}
public void testNestedAssign2() {
test("var a, b = a = 1; foo(b)",
"var b = 1; foo(b)");
}
public void testNestedAssign3() {
test("var a, b = a = 1; a = b = 2; foo(b)",
"var b = 1; b = 2; foo(b)");
}
public void testNestedAssign4() {
test("var a, b = a = 1; b = a = 2; foo(b)",
"var b = 1; b = 2; foo(b)");
}
public void testNestedAssign5() {
test("var a, b = a = 1; b = a = 2", "");
}
public void testNestedAssign15() {
test("var a, b, c; c = b = a = 2", "");
}
public void testNestedAssign6() {
testSame("var a, b, c; a = b = c = 1; foo(a, b, c)");
}
public void testNestedAssign7() {
testSame("var a = 0; a = i[j] = 1; b(a, i[j])");
}
public void testNestedAssign8() {
testSame("function f(){" +
"this.lockedToken_ = this.lastToken_ = " +
"SETPROP_value(this.hiddenInput_, a)}f()");
}
public void testRefChain1() {
test("var a = 1; var b = a; var c = b; var d = c", "");
}
public void testRefChain2() {
test("var a = 1; var b = a; var c = b; var d = c || f()",
"var a = 1; var b = a; var c = b; c || f()");
}
public void testRefChain3() {
test("var a = 1; var b = a; var c = b; var d = c + f()", "f()");
}
public void testRefChain4() {
test("var a = 1; var b = a; var c = b; var d = f() || c",
"f()");
}
public void testRefChain5() {
test("var a = 1; var b = a; var c = b; var d = f() ? g() : c",
"f() && g()");
}
public void testRefChain6() {
test("var a = 1; var b = a; var c = b; var d = c ? f() : g()",
"var a = 1; var b = a; var c = b; c ? f() : g()");
}
public void testRefChain7() {
test("var a = 1; var b = a; var c = b; var d = (b + f()) ? g() : c",
"var a = 1; var b = a; (b+f()) && g()");
}
public void testRefChain8() {
test("var a = 1; var b = a; var c = b; var d = f()[b] ? g() : 0",
"var a = 1; var b = a; f()[b] && g()");
}
public void testRefChain9() {
test("var a = 1; var b = a; var c = 5; var d = f()[b+c] ? g() : 0",
"var a = 1; var b = a; var c = 5; f()[b+c] && g()");
}
public void testRefChain10() {
test("var a = 1; var b = a; var c = b; var d = f()[b] ? g() : 0",
"var a = 1; var b = a; f()[b] && g()");
}
public void testRefChain11() {
test("var a = 1; var b = a; var d = f()[b] ? g() : 0",
"var a = 1; var b = a; f()[b] && g()");
}
public void testRefChain12() {
testSame("var a = 1; var b = a; f()[b] ? g() : 0");
}
public void testRefChain13() {
test("function f(){}var a = 1; var b = a; var d = f()[b] ? g() : 0",
"function f(){}var a = 1; var b = a; f()[b] && g()");
}
public void testRefChain14() {
testSame("function f(){}var a = 1; var b = a; f()[b] ? g() : 0");
}
public void testRefChain15() {
test("function f(){}var a = 1, b = a; var c = f(); var d = c[b] ? g() : 0",
"function f(){}var a = 1, b = a; var c = f(); c[b] && g()");
}
public void testRefChain16() {
testSame("function f(){}var a = 1; var b = a; var c = f(); c[b] ? g() : 0");
}
public void testRefChain17() {
test("function f(){}var a = 1; var b = a; var c = f(); var d = c[b]",
"function f(){} f()");
}
public void testRefChain18() {
testSame("var a = 1; f()[a] && g()");
}
public void testRefChain19() {
test("var a = 1; var b = [a]; var c = b; b[f()] ? g() : 0",
"var a=1; var b=[a]; b[f()] ? g() : 0");
}
public void testRefChain20() {
test("var a = 1; var b = [a]; var c = b; var d = b[f()] ? g() : 0",
"var a=1; var b=[a]; b[f()]&&g()");
}
public void testRefChain21() {
testSame("var a = 1; var b = 2; var c = a + b; f(c)");
}
public void testRefChain22() {
test("var a = 2; var b = a = 4; f(a)", "var a = 2; a = 4; f(a)");
}
public void testRefChain23() {
test("var a = {}; var b = a[1] || f()", "var a = {}; a[1] || f()");
}
/**
* Expressions that cannot be attributed to any enclosing dependency
* scope should be treated as global references.
* @bug 1739062
*/
public void testAssignmentWithComplexLhs() {
testSame("function f() { return this; }" +
"var o = {'key': 'val'};" +
"f().x_ = o['key'];");
}
public void testAssignmentWithComplexLhs2() {
testSame("function f() { return this; }" +
"var o = {'key': 'val'};" +
"f().foo = function() {" +
" o" +
"};");
}
public void testAssignmentWithComplexLhs3() {
String source =
"var o = {'key': 'val'};" +
"function init_() {" +
" this.x = o['key']" +
"}";
test(source, "");
testSame(source + ";init_()");
}
public void testAssignmentWithComplexLhs4() {
testSame("function f() { return this; }" +
"var o = {'key': 'val'};" +
"f().foo = function() {" +
" this.x = o['key']" +
"};");
}
/**
* Do not "prototype" property of variables that are not being
* tracked (because they are local).
* @bug 1809442
*/
public void testNoRemovePrototypeDefinitionsOutsideGlobalScope1() {
testSame("function f(arg){}" +
"" +
"(function(){" +
" var O = {};" +
" O.prototype = 'foo';" +
" f(O);" +
"})()");
}
public void testNoRemovePrototypeDefinitionsOutsideGlobalScope2() {
testSame("function f(arg){}" +
"(function h(){" +
" var L = {};" +
" L.prototype = 'foo';" +
" f(L);" +
"})()");
}
public void testNoRemovePrototypeDefinitionsOutsideGlobalScope4() {
testSame("function f(arg){}" +
"function g(){" +
" var N = {};" +
" N.prototype = 'foo';" +
" f(N);" +
"}" +
"g()");
}
public void testNoRemovePrototypeDefinitionsOutsideGlobalScope5() {
// function body not removed due to @bug 1898561
testSame("function g(){ var R = {}; R.prototype = 'foo' } g()");
}
public void testRemovePrototypeDefinitionsInGlobalScope1() {
testSame("function f(arg){}" +
"var M = {};" +
"M.prototype = 'foo';" +
"f(M);");
}
public void testRemovePrototypeDefinitionsInGlobalScope2() {
test("var Q = {}; Q.prototype = 'foo'", "");
}
public void testRemoveLabeledStatment() {
test("LBL: var x = 1;", "LBL: {}");
}
public void testRemoveLabeledStatment2() {
test("var x; LBL: x = f() + g()", "LBL: { f() ; g()}");
}
public void testRemoveLabeledStatment3() {
test("var x; LBL: x = 1;", "LBL: {}");
}
public void testRemoveLabeledStatment4() {
test("var a; LBL: a = f()", "LBL: f()");
}
public void testPreservePropertyMutationsToAlias1() {
// Test for issue b/2316773 - property get case
// Since a is referenced, property mutations via a's alias b must
// be preserved.
testSame("var a = {}; var b = a; b.x = 1; a");
}
public void testPreservePropertyMutationsToAlias2() {
// Test for issue b/2316773 - property get case, don't keep 'c'
test("var a = {}; var b = a; var c = a; b.x = 1; a",
"var a = {}; var b = a; b.x = 1; a");
}
public void testPreservePropertyMutationsToAlias3() {
// Test for issue b/2316773 - property get case, chain
testSame("var a = {}; var b = a; var c = b; c.x = 1; a");
}
public void testPreservePropertyMutationsToAlias4() {
// Test for issue b/2316773 - element get case
testSame("var a = {}; var b = a; b['x'] = 1; a");
}
public void testPreservePropertyMutationsToAlias5() {
// From issue b/2316773 description
testSame("function testCall(o){}" +
"var DATA = {'prop': 'foo','attr': {}};" +
"var SUBDATA = DATA['attr'];" +
"SUBDATA['subprop'] = 'bar';" +
"testCall(DATA);");
}
public void testPreservePropertyMutationsToAlias6() {
// Longer GETELEM chain
testSame("function testCall(o){}" +
"var DATA = {'prop': 'foo','attr': {}};" +
"var SUBDATA = DATA['attr'];" +
"var SUBSUBDATA = SUBDATA['subprop'];" +
"SUBSUBDATA['subsubprop'] = 'bar';" +
"testCall(DATA);");
}
public void testPreservePropertyMutationsToAlias7() {
// Make sure that the base class does not depend on the derived class.
test("var a = {}; var b = {}; b.x = 0;" +
"var goog = {}; goog.inherits(b, a); a",
"var a = {}; a");
}
public void testPreservePropertyMutationsToAlias8() {
// Make sure that the derived classes don't end up depending on each other.
test("var a = {};" +
"var b = {}; b.x = 0;" +
"var c = {}; c.y = 0;" +
"var goog = {}; goog.inherits(b, a); goog.inherits(c, a); c",
"var a = {}; var c = {}; c.y = 0;" +
"var goog = {}; goog.inherits(c, a); c");
}
public void testPreservePropertyMutationsToAlias9() {
testSame("var a = {b: {}};" +
"var c = a.b; c.d = 3;" +
"a.d = 3; a.d;");
}
public void testRemoveAlias() {
test("var a = {b: {}};" +
"var c = a.b;" +
"a.d = 3; a.d;",
"var a = {b: {}}; a.d = 3; a.d;");
}
public void testSingletonGetter1() {
test("function Foo() {} goog.addSingletonGetter(Foo);", "");
}
public void testSingletonGetter2() {
test("function Foo() {} goog$addSingletonGetter(Foo);", "");
}
public void testSingletonGetter3() {
// addSingletonGetter adds a getInstance method to a class.
testSame("function Foo() {} goog$addSingletonGetter(Foo);" +
"this.x = Foo.getInstance();");
}
public void testNoRemoveWindowPropertyAlias1() {
testSame(
"var self_ = window.gbar;\n" +
"self_.qs = function() {};");
}
public void testNoRemoveWindowPropertyAlias2() {
testSame(
"var self_ = window;\n" +
"self_.qs = function() {};");
}
public void testNoRemoveWindowPropertyAlias3() {
testSame(
"var self_ = window;\n" +
"self_['qs'] = function() {};");
}
public void testNoRemoveWindowPropertyAlias4() {
// TODO(johnlenz): fix this. "self_" should remain.
test(
"var self_ = window['gbar'] || {};\n" +
"self_.qs = function() {};",
"");
}
public void testNoRemoveWindowPropertyAlias4a() {
// TODO(johnlenz): fix this. "self_" should remain.
test(
"var self_; self_ = window.gbar || {};\n" +
"self_.qs = function() {};",
"");
}
public void testNoRemoveWindowPropertyAlias5() {
// TODO(johnlenz): fix this. "self_" should remain.
test(
"var self_ = window || {};\n" +
"self_['qs'] = function() {};",
"");
}
public void testNoRemoveWindowPropertyAlias5a() {
// TODO(johnlenz): fix this.
test(
"var self_; self_ = window || {};\n" +
"self_['qs'] = function() {};",
"");
}
public void testNoRemoveWindowPropertyAlias6() {
testSame(
"var self_ = (window.gbar = window.gbar || {});\n" +
"self_.qs = function() {};");
}
public void testNoRemoveWindowPropertyAlias6a() {
testSame(
"var self_; self_ = (window.gbar = window.gbar || {});\n" +
"self_.qs = function() {};");
}
public void testNoRemoveWindowPropertyAlias7() {
testSame(
"var self_ = (window = window || {});\n" +
"self_['qs'] = function() {};");
}
public void testNoRemoveWindowPropertyAlias7a() {
testSame(
"var self_; self_ = (window = window || {});\n" +
"self_['qs'] = function() {};");
}
public void testNoRemoveAlias0() {
testSame(
"var x = {}; function f() { return x; }; " +
"f().style.display = 'block';" +
"alert(x.style)");
}
public void testNoRemoveAlias1() {
testSame(
"var x = {}; function f() { return x; };" +
"var map = f();\n" +
"map.style.display = 'block';" +
"alert(x.style)");
}
public void testNoRemoveAlias2() {
testSame(
"var x = {};" +
"var map = (function () { return x; })();\n" +
"map.style = 'block';" +
"alert(x.style)");
}
public void testNoRemoveAlias3() {
testSame(
"var x = {}; function f() { return x; };" +
"var map = {}\n" +
"map[1] = f();\n" +
"map[1].style.display = 'block';");
}
public void testNoRemoveAliasOfExternal0() {
testSame(
"document.getElementById('foo').style.display = 'block';");
}
public void testNoRemoveAliasOfExternal1() {
testSame(
"var map = document.getElementById('foo');\n" +
"map.style.display = 'block';");
}
public void testNoRemoveAliasOfExternal2() {
testSame(
"var map = {}\n" +
"map[1] = document.getElementById('foo');\n" +
"map[1].style.display = 'block';");
}
public void testNoRemoveThrowReference1() {
testSame(
"var e = {}\n" +
"throw e;");
}
public void testNoRemoveThrowReference2() {
testSame(
"function e() {}\n" +
"throw new e();");
}
public void testClassDefinedInObjectLit1() {
test(
"var data = {Foo: function() {}};" +
"data.Foo.prototype.toString = function() {};",
"");
}
public void testClassDefinedInObjectLit2() {
test(
"var data = {}; data.bar = {Foo: function() {}};" +
"data.bar.Foo.prototype.toString = function() {};",
"");
}
public void testClassDefinedInObjectLit3() {
test(
"var data = {bar: {Foo: function() {}}};" +
"data.bar.Foo.prototype.toString = function() {};",
"");
}
public void testClassDefinedInObjectLit4() {
test(
"var data = {};" +
"data.baz = {bar: {Foo: function() {}}};" +
"data.baz.bar.Foo.prototype.toString = function() {};",
"");
}
public void testVarReferencedInClassDefinedInObjectLit1() {
testSame(
"var ref = 3;" +
"var data = {Foo: function() { this.x = ref; }};" +
"window.Foo = data.Foo;");
}
public void testVarReferencedInClassDefinedInObjectLit2() {
testSame(
"var ref = 3;" +
"var data = {Foo: function() { this.x = ref; }," +
" Bar: function() {}};" +
"window.Bar = data.Bar;");
}
public void testArrayExt() {
testSame(
"Array.prototype.foo = function() { return 1 };" +
"var y = [];" +
"switch (y.foo()) {" +
"}");
}
public void testArrayAliasExt() {
testSame(
"Array$X = Array;" +
"Array$X.prototype.foo = function() { return 1 };" +
"function Array$X() {}" +
"var y = [];" +
"switch (y.foo()) {" +
"}");
}
public void testExternalAliasInstanceof1() {
test(
"Array$X = Array;" +
"function Array$X() {}" +
"var y = [];" +
"if (y instanceof Array) {}",
"var y = [];" +
"if (y instanceof Array) {}"
);
}
public void testExternalAliasInstanceof2() {
testSame(
"Array$X = Array;" +
"function Array$X() {}" +
"var y = [];" +
"if (y instanceof Array$X) {}");
}
public void testExternalAliasInstanceof3() {
testSame(
"var b = Array;" +
"var y = [];" +
"if (y instanceof b) {}");
}
public void testAliasInstanceof4() {
testSame(
"function Foo() {};" +
"var b = Foo;" +
"var y = new Foo();" +
"if (y instanceof b) {}");
}
public void testAliasInstanceof5() {
// TODO(johnlenz): fix this. "b" should remain.
test(
"function Foo() {}" +
"function Bar() {}" +
"var b = x ? Foo : Bar;" +
"var y = new Foo();" +
"if (y instanceof b) {}",
"function Foo() {}" +
"var y = new Foo;" +
"if (false){}");
}
// We cannot leave x.a.prototype there because it will
// fail sanity var check.
public void testBrokenNamespaceWithPrototypeAssignment() {
test("var x = {}; x.a.prototype = 1", "");
}
public void testRemovePrototypeAliases() {
test(
"function g() {}" +
"function F() {} F.prototype.bar = g;" +
"window.g = g;",
"function g() {}" +
"window.g = g;");
}
public void testIssue284() {
test(
"var goog = {};" +
"goog.inherits = function(x, y) {};" +
"var ns = {};" +
"/** @constructor */" +
"ns.PageSelectionModel = function() {};" +
"/** @constructor */" +
"ns.PageSelectionModel.FooEvent = function() {};" +
"/** @constructor */" +
"ns.PageSelectionModel.SelectEvent = function() {};" +
"goog.inherits(ns.PageSelectionModel.ChangeEvent," +
" ns.PageSelectionModel.FooEvent);",
"");
}
public void testIssue838a() {
testSame("var z = window['z'] || (window['z'] = {});\n" +
"z['hello'] = 'Hello';\n" +
"z['world'] = 'World';");
}
public void testIssue838b() {
testSame(
"var z;" +
"window['z'] = z || (z = {});\n" +
"z['hello'] = 'Hello';\n" +
"z['world'] = 'World';");
}
public void testIssue874a() {
testSame(
"var a = a || {};\n" +
"var b = a;\n" +
"b.View = b.View || {}\n" +
"var c = b.View;\n" +
"c.Editor = function f(d, e) {\n" +
" return d + e\n" +
"};\n" +
"window.ImageEditor.View.Editor = a.View.Editor;");
}
public void testIssue874b() {
testSame(
"var b;\n" +
"var c = b = {};\n" +
"c.Editor = function f(d, e) {\n" +
" return d + e\n" +
"};\n" +
"window['Editor'] = b.Editor;");
}
public void testIssue874c() {
testSame(
"var b, c;\n" +
"c = b = {};\n" +
"c.Editor = function f(d, e) {\n" +
" return d + e\n" +
"};\n" +
"window['Editor'] = b.Editor;");
}
public void testIssue874d() {
testSame(
"var b = {}, c;\n" +
"c = b;\n" +
"c.Editor = function f(d, e) {\n" +
" return d + e\n" +
"};\n" +
"window['Editor'] = b.Editor;");
}
public void testIssue874e() {
testSame(
"var a;\n" +
"var b = a || (a = {});\n" +
"var c = b.View || (b.View = {});\n" +
"c.Editor = function f(d, e) {\n" +
" return d + e\n" +
"};\n" +
"window.ImageEditor.View.Editor = a.View.Editor;");
}
public void testBug6575051() {
testSame(
"var hackhack = window['__o_o_o__'] = window['__o_o_o__'] || {};\n" +
"window['__o_o_o__']['va'] = 1;\n" +
"hackhack['Vb'] = 1;");
}
@Override
protected CompilerPass getProcessor(Compiler compiler) {
return new MarkNoSideEffectCallsAndNameAnalyzerRunner(compiler);
}
private class MarkNoSideEffectCallsAndNameAnalyzerRunner
implements CompilerPass {
MarkNoSideEffectCalls markNoSideEffectCalls;
NameAnalyzer analyzer;
MarkNoSideEffectCallsAndNameAnalyzerRunner(Compiler compiler) {
this.markNoSideEffectCalls = new MarkNoSideEffectCalls(compiler);
this.analyzer = new NameAnalyzer(compiler, true);
}
@Override
public void process(Node externs, Node root) {
markNoSideEffectCalls.process(externs, root);
analyzer.process(externs, root);
}
}
} |
||
public void testImplementsExtendsLoop() throws Exception {
testClosureTypesMultipleWarnings(
suppressMissingProperty("foo") +
"/** @constructor \n * @implements {F} */var G = function() {};" +
"/** @constructor \n * @extends {G} */var F = function() {};" +
"alert((new F).foo);",
Lists.newArrayList(
"Parse error. Cycle detected in inheritance chain of type F"));
} | com.google.javascript.jscomp.TypeCheckTest::testImplementsExtendsLoop | test/com/google/javascript/jscomp/TypeCheckTest.java | 9,238 | test/com/google/javascript/jscomp/TypeCheckTest.java | testImplementsExtendsLoop | /*
* Copyright 2006 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import com.google.common.base.Joiner;
import com.google.common.collect.Lists;
import com.google.common.collect.Sets;
import com.google.javascript.jscomp.Scope.Var;
import com.google.javascript.jscomp.type.ClosureReverseAbstractInterpreter;
import com.google.javascript.jscomp.type.SemanticReverseAbstractInterpreter;
import com.google.javascript.rhino.InputId;
import com.google.javascript.rhino.Node;
import com.google.javascript.rhino.Token;
import com.google.javascript.rhino.jstype.FunctionType;
import com.google.javascript.rhino.jstype.JSType;
import com.google.javascript.rhino.jstype.JSTypeNative;
import com.google.javascript.rhino.jstype.ObjectType;
import com.google.javascript.rhino.testing.Asserts;
import java.util.Arrays;
import java.util.List;
import java.util.Set;
/**
* Tests {@link TypeCheck}.
*
*/
public class TypeCheckTest extends CompilerTypeTestCase {
private CheckLevel reportMissingOverrides = CheckLevel.WARNING;
@Override
public void setUp() throws Exception {
super.setUp();
reportMissingOverrides = CheckLevel.WARNING;
}
public void testInitialTypingScope() {
Scope s = new TypedScopeCreator(compiler,
CodingConventions.getDefault()).createInitialScope(
new Node(Token.BLOCK));
assertTypeEquals(ARRAY_FUNCTION_TYPE, s.getVar("Array").getType());
assertTypeEquals(BOOLEAN_OBJECT_FUNCTION_TYPE,
s.getVar("Boolean").getType());
assertTypeEquals(DATE_FUNCTION_TYPE, s.getVar("Date").getType());
assertTypeEquals(ERROR_FUNCTION_TYPE, s.getVar("Error").getType());
assertTypeEquals(EVAL_ERROR_FUNCTION_TYPE,
s.getVar("EvalError").getType());
assertTypeEquals(NUMBER_OBJECT_FUNCTION_TYPE,
s.getVar("Number").getType());
assertTypeEquals(OBJECT_FUNCTION_TYPE, s.getVar("Object").getType());
assertTypeEquals(RANGE_ERROR_FUNCTION_TYPE,
s.getVar("RangeError").getType());
assertTypeEquals(REFERENCE_ERROR_FUNCTION_TYPE,
s.getVar("ReferenceError").getType());
assertTypeEquals(REGEXP_FUNCTION_TYPE, s.getVar("RegExp").getType());
assertTypeEquals(STRING_OBJECT_FUNCTION_TYPE,
s.getVar("String").getType());
assertTypeEquals(SYNTAX_ERROR_FUNCTION_TYPE,
s.getVar("SyntaxError").getType());
assertTypeEquals(TYPE_ERROR_FUNCTION_TYPE,
s.getVar("TypeError").getType());
assertTypeEquals(URI_ERROR_FUNCTION_TYPE,
s.getVar("URIError").getType());
}
public void testPrivateType() throws Exception {
testTypes(
"/** @private {number} */ var x = false;",
"initializing variable\n" +
"found : boolean\n" +
"required: number");
}
public void testTypeCheck1() throws Exception {
testTypes("/**@return {void}*/function foo(){ if (foo()) return; }");
}
public void testTypeCheck2() throws Exception {
testTypes("/**@return {void}*/function foo(){ var x=foo(); x--; }",
"increment/decrement\n" +
"found : undefined\n" +
"required: number");
}
public void testTypeCheck4() throws Exception {
testTypes("/**@return {void}*/function foo(){ !foo(); }");
}
public void testTypeCheck5() throws Exception {
testTypes("/**@return {void}*/function foo(){ var a = +foo(); }",
"sign operator\n" +
"found : undefined\n" +
"required: number");
}
public void testTypeCheck6() throws Exception {
testTypes(
"/**@return {void}*/function foo(){" +
"/** @type {undefined|number} */var a;if (a == foo())return;}");
}
public void testTypeCheck8() throws Exception {
testTypes("/**@return {void}*/function foo(){do {} while (foo());}");
}
public void testTypeCheck9() throws Exception {
testTypes("/**@return {void}*/function foo(){while (foo());}");
}
public void testTypeCheck10() throws Exception {
testTypes("/**@return {void}*/function foo(){for (;foo(););}");
}
public void testTypeCheck11() throws Exception {
testTypes("/**@type !Number */var a;" +
"/**@type !String */var b;" +
"a = b;",
"assignment\n" +
"found : String\n" +
"required: Number");
}
public void testTypeCheck12() throws Exception {
testTypes("/**@return {!Object}*/function foo(){var a = 3^foo();}",
"bad right operand to bitwise operator\n" +
"found : Object\n" +
"required: (boolean|null|number|string|undefined)");
}
public void testTypeCheck13() throws Exception {
testTypes("/**@type {!Number|!String}*/var i; i=/xx/;",
"assignment\n" +
"found : RegExp\n" +
"required: (Number|String)");
}
public void testTypeCheck14() throws Exception {
testTypes("/**@param opt_a*/function foo(opt_a){}");
}
public void testTypeCheck15() throws Exception {
testTypes("/**@type {Number|null} */var x;x=null;x=10;",
"assignment\n" +
"found : number\n" +
"required: (Number|null)");
}
public void testTypeCheck16() throws Exception {
testTypes("/**@type {Number|null} */var x='';",
"initializing variable\n" +
"found : string\n" +
"required: (Number|null)");
}
public void testTypeCheck17() throws Exception {
testTypes("/**@return {Number}\n@param {Number} opt_foo */\n" +
"function a(opt_foo){\nreturn /**@type {Number}*/(opt_foo);\n}");
}
public void testTypeCheck18() throws Exception {
testTypes("/**@return {RegExp}\n*/\n function a(){return new RegExp();}");
}
public void testTypeCheck19() throws Exception {
testTypes("/**@return {Array}\n*/\n function a(){return new Array();}");
}
public void testTypeCheck20() throws Exception {
testTypes("/**@return {Date}\n*/\n function a(){return new Date();}");
}
public void testTypeCheckBasicDowncast() throws Exception {
testTypes("/** @constructor */function foo() {}\n" +
"/** @type {Object} */ var bar = new foo();\n");
}
public void testTypeCheckNoDowncastToNumber() throws Exception {
testTypes("/** @constructor */function foo() {}\n" +
"/** @type {!Number} */ var bar = new foo();\n",
"initializing variable\n" +
"found : foo\n" +
"required: Number");
}
public void testTypeCheck21() throws Exception {
testTypes("/** @type Array.<String> */var foo;");
}
public void testTypeCheck22() throws Exception {
testTypes("/** @param {Element|Object} p */\nfunction foo(p){}\n" +
"/** @constructor */function Element(){}\n" +
"/** @type {Element|Object} */var v;\n" +
"foo(v);\n");
}
public void testTypeCheck23() throws Exception {
testTypes("/** @type {(Object,Null)} */var foo; foo = null;");
}
public void testTypeCheck24() throws Exception {
testTypes("/** @constructor */function MyType(){}\n" +
"/** @type {(MyType,Null)} */var foo; foo = null;");
}
public void testTypeCheckDefaultExterns() throws Exception {
testTypes("/** @param {string} x */ function f(x) {}" +
"f([].length);" ,
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testTypeCheckCustomExterns() throws Exception {
testTypes(
DEFAULT_EXTERNS + "/** @type {boolean} */ Array.prototype.oogabooga;",
"/** @param {string} x */ function f(x) {}" +
"f([].oogabooga);" ,
"actual parameter 1 of f does not match formal parameter\n" +
"found : boolean\n" +
"required: string", false);
}
public void testTypeCheckCustomExterns2() throws Exception {
testTypes(
DEFAULT_EXTERNS + "/** @enum {string} */ var Enum = {FOO: 1, BAR: 1};",
"/** @param {Enum} x */ function f(x) {} f(Enum.FOO); f(true);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : boolean\n" +
"required: Enum.<string>",
false);
}
public void testParameterizedArray1() throws Exception {
testTypes("/** @param {!Array.<number>} a\n" +
"* @return {string}\n" +
"*/ var f = function(a) { return a[0]; };",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testParameterizedArray2() throws Exception {
testTypes("/** @param {!Array.<!Array.<number>>} a\n" +
"* @return {number}\n" +
"*/ var f = function(a) { return a[0]; };",
"inconsistent return type\n" +
"found : Array.<number>\n" +
"required: number");
}
public void testParameterizedArray3() throws Exception {
testTypes("/** @param {!Array.<number>} a\n" +
"* @return {number}\n" +
"*/ var f = function(a) { a[1] = 0; return a[0]; };");
}
public void testParameterizedArray4() throws Exception {
testTypes("/** @param {!Array.<number>} a\n" +
"*/ var f = function(a) { a[0] = 'a'; };",
"assignment\n" +
"found : string\n" +
"required: number");
}
public void testParameterizedArray5() throws Exception {
testTypes("/** @param {!Array.<*>} a\n" +
"*/ var f = function(a) { a[0] = 'a'; };");
}
public void testParameterizedArray6() throws Exception {
testTypes("/** @param {!Array.<*>} a\n" +
"* @return {string}\n" +
"*/ var f = function(a) { return a[0]; };",
"inconsistent return type\n" +
"found : *\n" +
"required: string");
}
public void testParameterizedArray7() throws Exception {
testTypes("/** @param {?Array.<number>} a\n" +
"* @return {string}\n" +
"*/ var f = function(a) { return a[0]; };",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testParameterizedObject1() throws Exception {
testTypes("/** @param {!Object.<number>} a\n" +
"* @return {string}\n" +
"*/ var f = function(a) { return a[0]; };",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testParameterizedObject2() throws Exception {
testTypes("/** @param {!Object.<string,number>} a\n" +
"* @return {string}\n" +
"*/ var f = function(a) { return a['x']; };",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testParameterizedObject3() throws Exception {
testTypes("/** @param {!Object.<number,string>} a\n" +
"* @return {string}\n" +
"*/ var f = function(a) { return a['x']; };",
"restricted index type\n" +
"found : string\n" +
"required: number");
}
public void testParameterizedObject4() throws Exception {
testTypes("/** @enum {string} */ var E = {A: 'a', B: 'b'};\n" +
"/** @param {!Object.<E,string>} a\n" +
"* @return {string}\n" +
"*/ var f = function(a) { return a['x']; };",
"restricted index type\n" +
"found : string\n" +
"required: E.<string>");
}
public void testParameterizedObject5() throws Exception {
testTypes("/** @constructor */ function F() {" +
" /** @type {Object.<number, string>} */ this.numbers = {};" +
"}" +
"(new F()).numbers['ten'] = '10';",
"restricted index type\n" +
"found : string\n" +
"required: number");
}
public void testUnionOfFunctionAndType() throws Exception {
testTypes("/** @type {null|(function(Number):void)} */ var a;" +
"/** @type {(function(Number):void)|null} */ var b = null; a = b;");
}
public void testOptionalParameterComparedToUndefined() throws Exception {
testTypes("/**@param opt_a {Number}*/function foo(opt_a)" +
"{if (opt_a==undefined) var b = 3;}");
}
public void testOptionalAllType() throws Exception {
testTypes("/** @param {*} opt_x */function f(opt_x) { return opt_x }\n" +
"/** @type {*} */var y;\n" +
"f(y);");
}
public void testOptionalUnknownNamedType() throws Exception {
testTypes("/** @param {!T} opt_x\n@return {undefined} */\n" +
"function f(opt_x) { return opt_x; }\n" +
"/** @constructor */var T = function() {};",
"inconsistent return type\n" +
"found : (T|undefined)\n" +
"required: undefined");
}
public void testOptionalArgFunctionParam() throws Exception {
testTypes("/** @param {function(number=)} a */" +
"function f(a) {a()};");
}
public void testOptionalArgFunctionParam2() throws Exception {
testTypes("/** @param {function(number=)} a */" +
"function f(a) {a(3)};");
}
public void testOptionalArgFunctionParam3() throws Exception {
testTypes("/** @param {function(number=)} a */" +
"function f(a) {a(undefined)};");
}
public void testOptionalArgFunctionParam4() throws Exception {
String expectedWarning = "Function a: called with 2 argument(s). " +
"Function requires at least 0 argument(s) and no more than 1 " +
"argument(s).";
testTypes("/** @param {function(number=)} a */function f(a) {a(3,4)};",
expectedWarning, false);
}
public void testOptionalArgFunctionParamError() throws Exception {
String expectedWarning =
"Bad type annotation. variable length argument must be last";
testTypes("/** @param {function(...[number], number=)} a */" +
"function f(a) {};", expectedWarning, false);
}
public void testOptionalNullableArgFunctionParam() throws Exception {
testTypes("/** @param {function(?number=)} a */" +
"function f(a) {a()};");
}
public void testOptionalNullableArgFunctionParam2() throws Exception {
testTypes("/** @param {function(?number=)} a */" +
"function f(a) {a(null)};");
}
public void testOptionalNullableArgFunctionParam3() throws Exception {
testTypes("/** @param {function(?number=)} a */" +
"function f(a) {a(3)};");
}
public void testOptionalArgFunctionReturn() throws Exception {
testTypes("/** @return {function(number=)} */" +
"function f() { return function(opt_x) { }; };" +
"f()()");
}
public void testOptionalArgFunctionReturn2() throws Exception {
testTypes("/** @return {function(Object=)} */" +
"function f() { return function(opt_x) { }; };" +
"f()({})");
}
public void testBooleanType() throws Exception {
testTypes("/**@type {boolean} */var x = 1 < 2;");
}
public void testBooleanReduction1() throws Exception {
testTypes("/**@type {string} */var x; x = null || \"a\";");
}
public void testBooleanReduction2() throws Exception {
// It's important for the type system to recognize that in no case
// can the boolean expression evaluate to a boolean value.
testTypes("/** @param {string} s\n @return {string} */" +
"(function(s) { return ((s == 'a') && s) || 'b'; })");
}
public void testBooleanReduction3() throws Exception {
testTypes("/** @param {string} s\n @return {string?} */" +
"(function(s) { return s && null && 3; })");
}
public void testBooleanReduction4() throws Exception {
testTypes("/** @param {Object} x\n @return {Object} */" +
"(function(x) { return null || x || null ; })");
}
public void testBooleanReduction5() throws Exception {
testTypes("/**\n" +
"* @param {Array|string} x\n" +
"* @return {string?}\n" +
"*/\n" +
"var f = function(x) {\n" +
"if (!x || typeof x == 'string') {\n" +
"return x;\n" +
"}\n" +
"return null;\n" +
"};");
}
public void testBooleanReduction6() throws Exception {
testTypes("/**\n" +
"* @param {Array|string|null} x\n" +
"* @return {string?}\n" +
"*/\n" +
"var f = function(x) {\n" +
"if (!(x && typeof x != 'string')) {\n" +
"return x;\n" +
"}\n" +
"return null;\n" +
"};");
}
public void testBooleanReduction7() throws Exception {
testTypes("/** @constructor */var T = function() {};\n" +
"/**\n" +
"* @param {Array|T} x\n" +
"* @return {null}\n" +
"*/\n" +
"var f = function(x) {\n" +
"if (!x) {\n" +
"return x;\n" +
"}\n" +
"return null;\n" +
"};");
}
public void testNullAnd() throws Exception {
testTypes("/** @type null */var x;\n" +
"/** @type number */var r = x && x;",
"initializing variable\n" +
"found : null\n" +
"required: number");
}
public void testNullOr() throws Exception {
testTypes("/** @type null */var x;\n" +
"/** @type number */var r = x || x;",
"initializing variable\n" +
"found : null\n" +
"required: number");
}
public void testBooleanPreservation1() throws Exception {
testTypes("/**@type {string} */var x = \"a\";" +
"x = ((x == \"a\") && x) || x == \"b\";",
"assignment\n" +
"found : (boolean|string)\n" +
"required: string");
}
public void testBooleanPreservation2() throws Exception {
testTypes("/**@type {string} */var x = \"a\"; x = (x == \"a\") || x;",
"assignment\n" +
"found : (boolean|string)\n" +
"required: string");
}
public void testBooleanPreservation3() throws Exception {
testTypes("/** @param {Function?} x\n @return {boolean?} */" +
"function f(x) { return x && x == \"a\"; }",
"condition always evaluates to false\n" +
"left : Function\n" +
"right: string");
}
public void testBooleanPreservation4() throws Exception {
testTypes("/** @param {Function?|boolean} x\n @return {boolean} */" +
"function f(x) { return x && x == \"a\"; }",
"inconsistent return type\n" +
"found : (boolean|null)\n" +
"required: boolean");
}
public void testTypeOfReduction1() throws Exception {
testTypes("/** @param {string|number} x\n @return {string} */ " +
"function f(x) { return typeof x == 'number' ? String(x) : x; }");
}
public void testTypeOfReduction2() throws Exception {
testTypes("/** @param {string|number} x\n @return {string} */ " +
"function f(x) { return typeof x != 'string' ? String(x) : x; }");
}
public void testTypeOfReduction3() throws Exception {
testTypes("/** @param {number|null} x\n @return {number} */ " +
"function f(x) { return typeof x == 'object' ? 1 : x; }");
}
public void testTypeOfReduction4() throws Exception {
testTypes("/** @param {Object|undefined} x\n @return {Object} */ " +
"function f(x) { return typeof x == 'undefined' ? {} : x; }");
}
public void testTypeOfReduction5() throws Exception {
testTypes("/** @enum {string} */ var E = {A: 'a', B: 'b'};\n" +
"/** @param {!E|number} x\n @return {string} */ " +
"function f(x) { return typeof x != 'number' ? x : 'a'; }");
}
public void testTypeOfReduction6() throws Exception {
testTypes("/** @param {number|string} x\n@return {string} */\n" +
"function f(x) {\n" +
"return typeof x == 'string' && x.length == 3 ? x : 'a';\n" +
"}");
}
public void testTypeOfReduction7() throws Exception {
testTypes("/** @return {string} */var f = function(x) { " +
"return typeof x == 'number' ? x : 'a'; }",
"inconsistent return type\n" +
"found : (number|string)\n" +
"required: string");
}
public void testTypeOfReduction8() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @param {number|string} x\n@return {string} */\n" +
"function f(x) {\n" +
"return goog.isString(x) && x.length == 3 ? x : 'a';\n" +
"}", null);
}
public void testTypeOfReduction9() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @param {!Array|string} x\n@return {string} */\n" +
"function f(x) {\n" +
"return goog.isArray(x) ? 'a' : x;\n" +
"}", null);
}
public void testTypeOfReduction10() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @param {Array|string} x\n@return {Array} */\n" +
"function f(x) {\n" +
"return goog.isArray(x) ? x : [];\n" +
"}", null);
}
public void testTypeOfReduction11() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @param {Array|string} x\n@return {Array} */\n" +
"function f(x) {\n" +
"return goog.isObject(x) ? x : [];\n" +
"}", null);
}
public void testTypeOfReduction12() throws Exception {
testTypes("/** @enum {string} */ var E = {A: 'a', B: 'b'};\n" +
"/** @param {E|Array} x\n @return {Array} */ " +
"function f(x) { return typeof x == 'object' ? x : []; }");
}
public void testTypeOfReduction13() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @enum {string} */ var E = {A: 'a', B: 'b'};\n" +
"/** @param {E|Array} x\n@return {Array} */ " +
"function f(x) { return goog.isObject(x) ? x : []; }", null);
}
public void testTypeOfReduction14() throws Exception {
// Don't do type inference on GETELEMs.
testClosureTypes(
CLOSURE_DEFS +
"function f(x) { " +
" return goog.isString(arguments[0]) ? arguments[0] : 0;" +
"}", null);
}
public void testTypeOfReduction15() throws Exception {
// Don't do type inference on GETELEMs.
testClosureTypes(
CLOSURE_DEFS +
"function f(x) { " +
" return typeof arguments[0] == 'string' ? arguments[0] : 0;" +
"}", null);
}
public void testTypeOfReduction16() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @interface */ function I() {}\n" +
"/**\n" +
" * @param {*} x\n" +
" * @return {I}\n" +
" */\n" +
"function f(x) { " +
" if(goog.isObject(x)) {" +
" return /** @type {I} */(x);" +
" }" +
" return null;" +
"}", null);
}
public void testQualifiedNameReduction1() throws Exception {
testTypes("var x = {}; /** @type {string?} */ x.a = 'a';\n" +
"/** @return {string} */ var f = function() {\n" +
"return x.a ? x.a : 'a'; }");
}
public void testQualifiedNameReduction2() throws Exception {
testTypes("/** @param {string?} a\n@constructor */ var T = " +
"function(a) {this.a = a};\n" +
"/** @return {string} */ T.prototype.f = function() {\n" +
"return this.a ? this.a : 'a'; }");
}
public void testQualifiedNameReduction3() throws Exception {
testTypes("/** @param {string|Array} a\n@constructor */ var T = " +
"function(a) {this.a = a};\n" +
"/** @return {string} */ T.prototype.f = function() {\n" +
"return typeof this.a == 'string' ? this.a : 'a'; }");
}
public void testQualifiedNameReduction4() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @param {string|Array} a\n@constructor */ var T = " +
"function(a) {this.a = a};\n" +
"/** @return {string} */ T.prototype.f = function() {\n" +
"return goog.isString(this.a) ? this.a : 'a'; }", null);
}
public void testQualifiedNameReduction5a() throws Exception {
testTypes("var x = {/** @type {string} */ a:'b' };\n" +
"/** @return {string} */ var f = function() {\n" +
"return x.a; }");
}
public void testQualifiedNameReduction5b() throws Exception {
testTypes(
"var x = {/** @type {number} */ a:12 };\n" +
"/** @return {string} */\n" +
"var f = function() {\n" +
" return x.a;\n" +
"}",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testQualifiedNameReduction5c() throws Exception {
testTypes(
"/** @return {string} */ var f = function() {\n" +
"var x = {/** @type {number} */ a:0 };\n" +
"return (x.a) ? (x.a) : 'a'; }",
"inconsistent return type\n" +
"found : (number|string)\n" +
"required: string");
}
public void testQualifiedNameReduction6() throws Exception {
testTypes(
"/** @return {string} */ var f = function() {\n" +
"var x = {/** @return {string?} */ get a() {return 'a'}};\n" +
"return x.a ? x.a : 'a'; }");
}
public void testQualifiedNameReduction7() throws Exception {
testTypes(
"/** @return {string} */ var f = function() {\n" +
"var x = {/** @return {number} */ get a() {return 12}};\n" +
"return x.a; }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testQualifiedNameReduction7a() throws Exception {
// It would be nice to find a way to make this an error.
testTypes(
"/** @return {string} */ var f = function() {\n" +
"var x = {get a() {return 12}};\n" +
"return x.a; }");
}
public void testQualifiedNameReduction8() throws Exception {
testTypes(
"/** @return {string} */ var f = function() {\n" +
"var x = {get a() {return 'a'}};\n" +
"return x.a ? x.a : 'a'; }");
}
public void testQualifiedNameReduction9() throws Exception {
testTypes(
"/** @return {string} */ var f = function() {\n" +
"var x = { /** @param {string} b */ set a(b) {}};\n" +
"return x.a ? x.a : 'a'; }");
}
public void testQualifiedNameReduction10() throws Exception {
// TODO(johnlenz): separate setter property types from getter property
// types.
testTypes(
"/** @return {string} */ var f = function() {\n" +
"var x = { /** @param {number} b */ set a(b) {}};\n" +
"return x.a ? x.a : 'a'; }",
"inconsistent return type\n" +
"found : (number|string)\n" +
"required: string");
}
public void testObjLitDef1a() throws Exception {
testTypes(
"var x = {/** @type {number} */ a:12 };\n" +
"x.a = 'a';",
"assignment to property a of x\n" +
"found : string\n" +
"required: number");
}
public void testObjLitDef1b() throws Exception {
testTypes(
"function f(){" +
"var x = {/** @type {number} */ a:12 };\n" +
"x.a = 'a';" +
"};\n" +
"f();",
"assignment to property a of x\n" +
"found : string\n" +
"required: number");
}
public void testObjLitDef2a() throws Exception {
testTypes(
"var x = {/** @param {number} b */ set a(b){} };\n" +
"x.a = 'a';",
"assignment to property a of x\n" +
"found : string\n" +
"required: number");
}
public void testObjLitDef2b() throws Exception {
testTypes(
"function f(){" +
"var x = {/** @param {number} b */ set a(b){} };\n" +
"x.a = 'a';" +
"};\n" +
"f();",
"assignment to property a of x\n" +
"found : string\n" +
"required: number");
}
public void testObjLitDef3a() throws Exception {
testTypes(
"/** @type {string} */ var y;\n" +
"var x = {/** @return {number} */ get a(){} };\n" +
"y = x.a;",
"assignment\n" +
"found : number\n" +
"required: string");
}
public void testObjLitDef3b() throws Exception {
testTypes(
"/** @type {string} */ var y;\n" +
"function f(){" +
"var x = {/** @return {number} */ get a(){} };\n" +
"y = x.a;" +
"};\n" +
"f();",
"assignment\n" +
"found : number\n" +
"required: string");
}
public void testObjLitDef4() throws Exception {
testTypes(
"var x = {" +
"/** @return {number} */ a:12 };\n",
"assignment to property a of {a: function (): number}\n" +
"found : number\n" +
"required: function (): number");
}
public void testObjLitDef5() throws Exception {
testTypes(
"var x = {};\n" +
"/** @return {number} */ x.a = 12;\n",
"assignment to property a of x\n" +
"found : number\n" +
"required: function (): number");
}
public void testInstanceOfReduction1() throws Exception {
testTypes("/** @constructor */ var T = function() {};\n" +
"/** @param {T|string} x\n@return {T} */\n" +
"var f = function(x) {\n" +
"if (x instanceof T) { return x; } else { return new T(); }\n" +
"};");
}
public void testInstanceOfReduction2() throws Exception {
testTypes("/** @constructor */ var T = function() {};\n" +
"/** @param {!T|string} x\n@return {string} */\n" +
"var f = function(x) {\n" +
"if (x instanceof T) { return ''; } else { return x; }\n" +
"};");
}
public void testUndeclaredGlobalProperty1() throws Exception {
testTypes("/** @const */ var x = {}; x.y = null;" +
"function f(a) { x.y = a; }" +
"/** @param {string} a */ function g(a) { }" +
"function h() { g(x.y); }");
}
public void testUndeclaredGlobalProperty2() throws Exception {
testTypes("/** @const */ var x = {}; x.y = null;" +
"function f() { x.y = 3; }" +
"/** @param {string} a */ function g(a) { }" +
"function h() { g(x.y); }",
"actual parameter 1 of g does not match formal parameter\n" +
"found : (null|number)\n" +
"required: string");
}
public void testLocallyInferredGlobalProperty1() throws Exception {
// We used to have a bug where x.y.z leaked from f into h.
testTypes(
"/** @constructor */ function F() {}" +
"/** @type {number} */ F.prototype.z;" +
"/** @const */ var x = {}; /** @type {F} */ x.y;" +
"function f() { x.y.z = 'abc'; }" +
"/** @param {number} x */ function g(x) {}" +
"function h() { g(x.y.z); }",
"assignment to property z of F\n" +
"found : string\n" +
"required: number");
}
public void testPropertyInferredPropagation() throws Exception {
testTypes("/** @return {Object} */function f() { return {}; }\n" +
"function g() { var x = f(); if (x.p) x.a = 'a'; else x.a = 'b'; }\n" +
"function h() { var x = f(); x.a = false; }");
}
public void testPropertyInference1() throws Exception {
testTypes(
"/** @constructor */ function F() { this.x_ = true; }" +
"/** @return {string} */" +
"F.prototype.bar = function() { if (this.x_) return this.x_; };",
"inconsistent return type\n" +
"found : boolean\n" +
"required: string");
}
public void testPropertyInference2() throws Exception {
testTypes(
"/** @constructor */ function F() { this.x_ = true; }" +
"F.prototype.baz = function() { this.x_ = null; };" +
"/** @return {string} */" +
"F.prototype.bar = function() { if (this.x_) return this.x_; };",
"inconsistent return type\n" +
"found : boolean\n" +
"required: string");
}
public void testPropertyInference3() throws Exception {
testTypes(
"/** @constructor */ function F() { this.x_ = true; }" +
"F.prototype.baz = function() { this.x_ = 3; };" +
"/** @return {string} */" +
"F.prototype.bar = function() { if (this.x_) return this.x_; };",
"inconsistent return type\n" +
"found : (boolean|number)\n" +
"required: string");
}
public void testPropertyInference4() throws Exception {
testTypes(
"/** @constructor */ function F() { }" +
"F.prototype.x_ = 3;" +
"/** @return {string} */" +
"F.prototype.bar = function() { if (this.x_) return this.x_; };",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testPropertyInference5() throws Exception {
testTypes(
"/** @constructor */ function F() { }" +
"F.prototype.baz = function() { this.x_ = 3; };" +
"/** @return {string} */" +
"F.prototype.bar = function() { if (this.x_) return this.x_; };");
}
public void testPropertyInference6() throws Exception {
testTypes(
"/** @constructor */ function F() { }" +
"(new F).x_ = 3;" +
"/** @return {string} */" +
"F.prototype.bar = function() { return this.x_; };");
}
public void testPropertyInference7() throws Exception {
testTypes(
"/** @constructor */ function F() { this.x_ = true; }" +
"(new F).x_ = 3;" +
"/** @return {string} */" +
"F.prototype.bar = function() { return this.x_; };",
"inconsistent return type\n" +
"found : boolean\n" +
"required: string");
}
public void testPropertyInference8() throws Exception {
testTypes(
"/** @constructor */ function F() { " +
" /** @type {string} */ this.x_ = 'x';" +
"}" +
"(new F).x_ = 3;" +
"/** @return {string} */" +
"F.prototype.bar = function() { return this.x_; };",
"assignment to property x_ of F\n" +
"found : number\n" +
"required: string");
}
public void testPropertyInference9() throws Exception {
testTypes(
"/** @constructor */ function A() {}" +
"/** @return {function(): ?} */ function f() { " +
" return function() {};" +
"}" +
"var g = f();" +
"/** @type {number} */ g.prototype.bar_ = null;",
"assignment\n" +
"found : null\n" +
"required: number");
}
public void testPropertyInference10() throws Exception {
// NOTE(nicksantos): There used to be a bug where a property
// on the prototype of one structural function would leak onto
// the prototype of other variables with the same structural
// function type.
testTypes(
"/** @constructor */ function A() {}" +
"/** @return {function(): ?} */ function f() { " +
" return function() {};" +
"}" +
"var g = f();" +
"/** @type {number} */ g.prototype.bar_ = 1;" +
"var h = f();" +
"/** @type {string} */ h.prototype.bar_ = 1;",
"assignment\n" +
"found : number\n" +
"required: string");
}
public void testNoPersistentTypeInferenceForObjectProperties()
throws Exception {
testTypes("/** @param {Object} o\n@param {string} x */\n" +
"function s1(o,x) { o.x = x; }\n" +
"/** @param {Object} o\n@return {string} */\n" +
"function g1(o) { return typeof o.x == 'undefined' ? '' : o.x; }\n" +
"/** @param {Object} o\n@param {number} x */\n" +
"function s2(o,x) { o.x = x; }\n" +
"/** @param {Object} o\n@return {number} */\n" +
"function g2(o) { return typeof o.x == 'undefined' ? 0 : o.x; }");
}
public void testNoPersistentTypeInferenceForFunctionProperties()
throws Exception {
testTypes("/** @param {Function} o\n@param {string} x */\n" +
"function s1(o,x) { o.x = x; }\n" +
"/** @param {Function} o\n@return {string} */\n" +
"function g1(o) { return typeof o.x == 'undefined' ? '' : o.x; }\n" +
"/** @param {Function} o\n@param {number} x */\n" +
"function s2(o,x) { o.x = x; }\n" +
"/** @param {Function} o\n@return {number} */\n" +
"function g2(o) { return typeof o.x == 'undefined' ? 0 : o.x; }");
}
public void testObjectPropertyTypeInferredInLocalScope1() throws Exception {
testTypes("/** @param {!Object} o\n@return {string} */\n" +
"function f(o) { o.x = 1; return o.x; }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testObjectPropertyTypeInferredInLocalScope2() throws Exception {
testTypes("/**@param {!Object} o\n@param {number?} x\n@return {string}*/" +
"function f(o, x) { o.x = 'a';\nif (x) {o.x = x;}\nreturn o.x; }",
"inconsistent return type\n" +
"found : (number|string)\n" +
"required: string");
}
public void testObjectPropertyTypeInferredInLocalScope3() throws Exception {
testTypes("/**@param {!Object} o\n@param {number?} x\n@return {string}*/" +
"function f(o, x) { if (x) {o.x = x;} else {o.x = 'a';}\nreturn o.x; }",
"inconsistent return type\n" +
"found : (number|string)\n" +
"required: string");
}
public void testMismatchingOverridingInferredPropertyBeforeDeclaredProperty1()
throws Exception {
testTypes("/** @constructor */var T = function() { this.x = ''; };\n" +
"/** @type {number} */ T.prototype.x = 0;",
"assignment to property x of T\n" +
"found : string\n" +
"required: number");
}
public void testMismatchingOverridingInferredPropertyBeforeDeclaredProperty2()
throws Exception {
testTypes("/** @constructor */var T = function() { this.x = ''; };\n" +
"/** @type {number} */ T.prototype.x;",
"assignment to property x of T\n" +
"found : string\n" +
"required: number");
}
public void testMismatchingOverridingInferredPropertyBeforeDeclaredProperty3()
throws Exception {
testTypes("/** @type {Object} */ var n = {};\n" +
"/** @constructor */ n.T = function() { this.x = ''; };\n" +
"/** @type {number} */ n.T.prototype.x = 0;",
"assignment to property x of n.T\n" +
"found : string\n" +
"required: number");
}
public void testMismatchingOverridingInferredPropertyBeforeDeclaredProperty4()
throws Exception {
testTypes("var n = {};\n" +
"/** @constructor */ n.T = function() { this.x = ''; };\n" +
"/** @type {number} */ n.T.prototype.x = 0;",
"assignment to property x of n.T\n" +
"found : string\n" +
"required: number");
}
public void testPropertyUsedBeforeDefinition1() throws Exception {
testTypes("/** @constructor */ var T = function() {};\n" +
"/** @return {string} */" +
"T.prototype.f = function() { return this.g(); };\n" +
"/** @return {number} */ T.prototype.g = function() { return 1; };\n",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testPropertyUsedBeforeDefinition2() throws Exception {
testTypes("var n = {};\n" +
"/** @constructor */ n.T = function() {};\n" +
"/** @return {string} */" +
"n.T.prototype.f = function() { return this.g(); };\n" +
"/** @return {number} */ n.T.prototype.g = function() { return 1; };\n",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testAdd1() throws Exception {
testTypes("/**@return {void}*/function foo(){var a = 'abc'+foo();}");
}
public void testAdd2() throws Exception {
testTypes("/**@return {void}*/function foo(){var a = foo()+4;}");
}
public void testAdd3() throws Exception {
testTypes("/** @type {string} */ var a = 'a';" +
"/** @type {string} */ var b = 'b';" +
"/** @type {string} */ var c = a + b;");
}
public void testAdd4() throws Exception {
testTypes("/** @type {number} */ var a = 5;" +
"/** @type {string} */ var b = 'b';" +
"/** @type {string} */ var c = a + b;");
}
public void testAdd5() throws Exception {
testTypes("/** @type {string} */ var a = 'a';" +
"/** @type {number} */ var b = 5;" +
"/** @type {string} */ var c = a + b;");
}
public void testAdd6() throws Exception {
testTypes("/** @type {number} */ var a = 5;" +
"/** @type {number} */ var b = 5;" +
"/** @type {number} */ var c = a + b;");
}
public void testAdd7() throws Exception {
testTypes("/** @type {number} */ var a = 5;" +
"/** @type {string} */ var b = 'b';" +
"/** @type {number} */ var c = a + b;",
"initializing variable\n" +
"found : string\n" +
"required: number");
}
public void testAdd8() throws Exception {
testTypes("/** @type {string} */ var a = 'a';" +
"/** @type {number} */ var b = 5;" +
"/** @type {number} */ var c = a + b;",
"initializing variable\n" +
"found : string\n" +
"required: number");
}
public void testAdd9() throws Exception {
testTypes("/** @type {number} */ var a = 5;" +
"/** @type {number} */ var b = 5;" +
"/** @type {string} */ var c = a + b;",
"initializing variable\n" +
"found : number\n" +
"required: string");
}
public void testAdd10() throws Exception {
// d.e.f will have unknown type.
testTypes(
suppressMissingProperty("e", "f") +
"/** @type {number} */ var a = 5;" +
"/** @type {string} */ var c = a + d.e.f;");
}
public void testAdd11() throws Exception {
// d.e.f will have unknown type.
testTypes(
suppressMissingProperty("e", "f") +
"/** @type {number} */ var a = 5;" +
"/** @type {number} */ var c = a + d.e.f;");
}
public void testAdd12() throws Exception {
testTypes("/** @return {(number,string)} */ function a() { return 5; }" +
"/** @type {number} */ var b = 5;" +
"/** @type {boolean} */ var c = a() + b;",
"initializing variable\n" +
"found : (number|string)\n" +
"required: boolean");
}
public void testAdd13() throws Exception {
testTypes("/** @type {number} */ var a = 5;" +
"/** @return {(number,string)} */ function b() { return 5; }" +
"/** @type {boolean} */ var c = a + b();",
"initializing variable\n" +
"found : (number|string)\n" +
"required: boolean");
}
public void testAdd14() throws Exception {
testTypes("/** @type {(null,string)} */ var a = null;" +
"/** @type {number} */ var b = 5;" +
"/** @type {boolean} */ var c = a + b;",
"initializing variable\n" +
"found : (number|string)\n" +
"required: boolean");
}
public void testAdd15() throws Exception {
testTypes("/** @type {number} */ var a = 5;" +
"/** @return {(number,string)} */ function b() { return 5; }" +
"/** @type {boolean} */ var c = a + b();",
"initializing variable\n" +
"found : (number|string)\n" +
"required: boolean");
}
public void testAdd16() throws Exception {
testTypes("/** @type {(undefined,string)} */ var a = undefined;" +
"/** @type {number} */ var b = 5;" +
"/** @type {boolean} */ var c = a + b;",
"initializing variable\n" +
"found : (number|string)\n" +
"required: boolean");
}
public void testAdd17() throws Exception {
testTypes("/** @type {number} */ var a = 5;" +
"/** @type {(undefined,string)} */ var b = undefined;" +
"/** @type {boolean} */ var c = a + b;",
"initializing variable\n" +
"found : (number|string)\n" +
"required: boolean");
}
public void testAdd18() throws Exception {
testTypes("function f() {};" +
"/** @type {string} */ var a = 'a';" +
"/** @type {number} */ var c = a + f();",
"initializing variable\n" +
"found : string\n" +
"required: number");
}
public void testAdd19() throws Exception {
testTypes("/** @param {number} opt_x\n@param {number} opt_y\n" +
"@return {number} */ function f(opt_x, opt_y) {" +
"return opt_x + opt_y;}");
}
public void testAdd20() throws Exception {
testTypes("/** @param {!Number} opt_x\n@param {!Number} opt_y\n" +
"@return {number} */ function f(opt_x, opt_y) {" +
"return opt_x + opt_y;}");
}
public void testAdd21() throws Exception {
testTypes("/** @param {Number|Boolean} opt_x\n" +
"@param {number|boolean} opt_y\n" +
"@return {number} */ function f(opt_x, opt_y) {" +
"return opt_x + opt_y;}");
}
public void testNumericComparison1() throws Exception {
testTypes("/**@param {number} a*/ function f(a) {return a < 3;}");
}
public void testNumericComparison2() throws Exception {
testTypes("/**@param {!Object} a*/ function f(a) {return a < 3;}",
"left side of numeric comparison\n" +
"found : Object\n" +
"required: number");
}
public void testNumericComparison3() throws Exception {
testTypes("/**@param {string} a*/ function f(a) {return a < 3;}");
}
public void testNumericComparison4() throws Exception {
testTypes("/**@param {(number,undefined)} a*/ " +
"function f(a) {return a < 3;}");
}
public void testNumericComparison5() throws Exception {
testTypes("/**@param {*} a*/ function f(a) {return a < 3;}",
"left side of numeric comparison\n" +
"found : *\n" +
"required: number");
}
public void testNumericComparison6() throws Exception {
testTypes("/**@return {void} */ function foo() { if (3 >= foo()) return; }",
"right side of numeric comparison\n" +
"found : undefined\n" +
"required: number");
}
public void testStringComparison1() throws Exception {
testTypes("/**@param {string} a*/ function f(a) {return a < 'x';}");
}
public void testStringComparison2() throws Exception {
testTypes("/**@param {Object} a*/ function f(a) {return a < 'x';}");
}
public void testStringComparison3() throws Exception {
testTypes("/**@param {number} a*/ function f(a) {return a < 'x';}");
}
public void testStringComparison4() throws Exception {
testTypes("/**@param {string|undefined} a*/ " +
"function f(a) {return a < 'x';}");
}
public void testStringComparison5() throws Exception {
testTypes("/**@param {*} a*/ " +
"function f(a) {return a < 'x';}");
}
public void testStringComparison6() throws Exception {
testTypes("/**@return {void} */ " +
"function foo() { if ('a' >= foo()) return; }",
"right side of comparison\n" +
"found : undefined\n" +
"required: string");
}
public void testValueOfComparison1() throws Exception {
testTypes("/** @constructor */function O() {};" +
"/**@override*/O.prototype.valueOf = function() { return 1; };" +
"/**@param {!O} a\n@param {!O} b*/ function f(a,b) { return a < b; }");
}
public void testValueOfComparison2() throws Exception {
testTypes("/** @constructor */function O() {};" +
"/**@override*/O.prototype.valueOf = function() { return 1; };" +
"/**@param {!O} a\n@param {number} b*/" +
"function f(a,b) { return a < b; }");
}
public void testValueOfComparison3() throws Exception {
testTypes("/** @constructor */function O() {};" +
"/**@override*/O.prototype.toString = function() { return 'o'; };" +
"/**@param {!O} a\n@param {string} b*/" +
"function f(a,b) { return a < b; }");
}
public void testGenericRelationalExpression() throws Exception {
testTypes("/**@param {*} a\n@param {*} b*/ " +
"function f(a,b) {return a < b;}");
}
public void testInstanceof1() throws Exception {
testTypes("function foo(){" +
"if (bar instanceof 3)return;}",
"instanceof requires an object\n" +
"found : number\n" +
"required: Object");
}
public void testInstanceof2() throws Exception {
testTypes("/**@return {void}*/function foo(){" +
"if (foo() instanceof Object)return;}",
"deterministic instanceof yields false\n" +
"found : undefined\n" +
"required: NoObject");
}
public void testInstanceof3() throws Exception {
testTypes("/**@return {*} */function foo(){" +
"if (foo() instanceof Object)return;}");
}
public void testInstanceof4() throws Exception {
testTypes("/**@return {(Object|number)} */function foo(){" +
"if (foo() instanceof Object)return 3;}");
}
public void testInstanceof5() throws Exception {
// No warning for unknown types.
testTypes("/** @return {?} */ function foo(){" +
"if (foo() instanceof Object)return;}");
}
public void testInstanceof6() throws Exception {
testTypes("/**@return {(Array|number)} */function foo(){" +
"if (foo() instanceof Object)return 3;}");
}
public void testInstanceOfReduction3() throws Exception {
testTypes(
"/** \n" +
" * @param {Object} x \n" +
" * @param {Function} y \n" +
" * @return {boolean} \n" +
" */\n" +
"var f = function(x, y) {\n" +
" return x instanceof y;\n" +
"};");
}
public void testScoping1() throws Exception {
testTypes(
"/**@param {string} a*/function foo(a){" +
" /**@param {Array|string} a*/function bar(a){" +
" if (a instanceof Array)return;" +
" }" +
"}");
}
public void testScoping2() throws Exception {
testTypes(
"/** @type number */ var a;" +
"function Foo() {" +
" /** @type string */ var a;" +
"}");
}
public void testScoping3() throws Exception {
testTypes("\n\n/** @type{Number}*/var b;\n/** @type{!String} */var b;",
"variable b redefined with type String, original " +
"definition at [testcode]:3 with type (Number|null)");
}
public void testScoping4() throws Exception {
testTypes("/** @type{Number}*/var b; if (true) /** @type{!String} */var b;",
"variable b redefined with type String, original " +
"definition at [testcode]:1 with type (Number|null)");
}
public void testScoping5() throws Exception {
// multiple definitions are not checked by the type checker but by a
// subsequent pass
testTypes("if (true) var b; var b;");
}
public void testScoping6() throws Exception {
// multiple definitions are not checked by the type checker but by a
// subsequent pass
testTypes("if (true) var b; if (true) var b;");
}
public void testScoping7() throws Exception {
testTypes("/** @constructor */function A() {" +
" /** @type !A */this.a = null;" +
"}",
"assignment to property a of A\n" +
"found : null\n" +
"required: A");
}
public void testScoping8() throws Exception {
testTypes("/** @constructor */function A() {}" +
"/** @constructor */function B() {" +
" /** @type !A */this.a = null;" +
"}",
"assignment to property a of B\n" +
"found : null\n" +
"required: A");
}
public void testScoping9() throws Exception {
testTypes("/** @constructor */function B() {" +
" /** @type !A */this.a = null;" +
"}" +
"/** @constructor */function A() {}",
"assignment to property a of B\n" +
"found : null\n" +
"required: A");
}
public void testScoping10() throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope("var a = function b(){};");
// a declared, b is not
assertTrue(p.scope.isDeclared("a", false));
assertFalse(p.scope.isDeclared("b", false));
// checking that a has the correct assigned type
assertEquals("function (): undefined",
p.scope.getVar("a").getType().toString());
}
public void testScoping11() throws Exception {
// named function expressions create a binding in their body only
// the return is wrong but the assignment is OK since the type of b is ?
testTypes(
"/** @return {number} */var a = function b(){ return b };",
"inconsistent return type\n" +
"found : function (): number\n" +
"required: number");
}
public void testScoping12() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** @type {number} */ F.prototype.bar = 3;" +
"/** @param {!F} f */ function g(f) {" +
" /** @return {string} */" +
" function h() {" +
" return f.bar;" +
" }" +
"}",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testFunctionArguments1() throws Exception {
testFunctionType(
"/** @param {number} a\n@return {string} */" +
"function f(a) {}",
"function (number): string");
}
public void testFunctionArguments2() throws Exception {
testFunctionType(
"/** @param {number} opt_a\n@return {string} */" +
"function f(opt_a) {}",
"function (number=): string");
}
public void testFunctionArguments3() throws Exception {
testFunctionType(
"/** @param {number} b\n@return {string} */" +
"function f(a,b) {}",
"function (?, number): string");
}
public void testFunctionArguments4() throws Exception {
testFunctionType(
"/** @param {number} opt_a\n@return {string} */" +
"function f(a,opt_a) {}",
"function (?, number=): string");
}
public void testFunctionArguments5() throws Exception {
testTypes(
"function a(opt_a,a) {}",
"optional arguments must be at the end");
}
public void testFunctionArguments6() throws Exception {
testTypes(
"function a(var_args,a) {}",
"variable length argument must be last");
}
public void testFunctionArguments7() throws Exception {
testTypes(
"/** @param {number} opt_a\n@return {string} */" +
"function a(a,opt_a,var_args) {}");
}
public void testFunctionArguments8() throws Exception {
testTypes(
"function a(a,opt_a,var_args,b) {}",
"variable length argument must be last");
}
public void testFunctionArguments9() throws Exception {
// testing that only one error is reported
testTypes(
"function a(a,opt_a,var_args,b,c) {}",
"variable length argument must be last");
}
public void testFunctionArguments10() throws Exception {
// testing that only one error is reported
testTypes(
"function a(a,opt_a,b,c) {}",
"optional arguments must be at the end");
}
public void testFunctionArguments11() throws Exception {
testTypes(
"function a(a,opt_a,b,c,var_args,d) {}",
"optional arguments must be at the end");
}
public void testFunctionArguments12() throws Exception {
testTypes("/** @param foo {String} */function bar(baz){}",
"parameter foo does not appear in bar's parameter list");
}
public void testFunctionArguments13() throws Exception {
// verifying that the argument type have non-inferable types
testTypes(
"/** @return {boolean} */ function u() { return true; }" +
"/** @param {boolean} b\n@return {?boolean} */" +
"function f(b) { if (u()) { b = null; } return b; }",
"assignment\n" +
"found : null\n" +
"required: boolean");
}
public void testFunctionArguments14() throws Exception {
testTypes(
"/**\n" +
" * @param {string} x\n" +
" * @param {number} opt_y\n" +
" * @param {boolean} var_args\n" +
" */ function f(x, opt_y, var_args) {}" +
"f('3'); f('3', 2); f('3', 2, true); f('3', 2, true, false);");
}
public void testFunctionArguments15() throws Exception {
testTypes(
"/** @param {?function(*)} f */" +
"function g(f) { f(1, 2); }",
"Function f: called with 2 argument(s). " +
"Function requires at least 1 argument(s) " +
"and no more than 1 argument(s).");
}
public void testFunctionArguments16() throws Exception {
testTypes(
"/** @param {...number} var_args */" +
"function g(var_args) {} g(1, true);",
"actual parameter 2 of g does not match formal parameter\n" +
"found : boolean\n" +
"required: (number|undefined)");
}
public void testFunctionArguments17() throws Exception {
testClosureTypesMultipleWarnings(
"/** @param {booool|string} x */" +
"function f(x) { g(x) }" +
"/** @param {number} x */" +
"function g(x) {}",
Lists.newArrayList(
"Bad type annotation. Unknown type booool",
"actual parameter 1 of g does not match formal parameter\n" +
"found : (booool|null|string)\n" +
"required: number"));
}
public void testPrintFunctionName1() throws Exception {
// Ensures that the function name is pretty.
testTypes(
"var goog = {}; goog.run = function(f) {};" +
"goog.run();",
"Function goog.run: called with 0 argument(s). " +
"Function requires at least 1 argument(s) " +
"and no more than 1 argument(s).");
}
public void testPrintFunctionName2() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {}; " +
"Foo.prototype.run = function(f) {};" +
"(new Foo).run();",
"Function Foo.prototype.run: called with 0 argument(s). " +
"Function requires at least 1 argument(s) " +
"and no more than 1 argument(s).");
}
public void testFunctionInference1() throws Exception {
testFunctionType(
"function f(a) {}",
"function (?): undefined");
}
public void testFunctionInference2() throws Exception {
testFunctionType(
"function f(a,b) {}",
"function (?, ?): undefined");
}
public void testFunctionInference3() throws Exception {
testFunctionType(
"function f(var_args) {}",
"function (...[?]): undefined");
}
public void testFunctionInference4() throws Exception {
testFunctionType(
"function f(a,b,c,var_args) {}",
"function (?, ?, ?, ...[?]): undefined");
}
public void testFunctionInference5() throws Exception {
testFunctionType(
"/** @this Date\n@return {string} */function f(a) {}",
"function (this:Date, ?): string");
}
public void testFunctionInference6() throws Exception {
testFunctionType(
"/** @this Date\n@return {string} */function f(opt_a) {}",
"function (this:Date, ?=): string");
}
public void testFunctionInference7() throws Exception {
testFunctionType(
"/** @this Date */function f(a,b,c,var_args) {}",
"function (this:Date, ?, ?, ?, ...[?]): undefined");
}
public void testFunctionInference8() throws Exception {
testFunctionType(
"function f() {}",
"function (): undefined");
}
public void testFunctionInference9() throws Exception {
testFunctionType(
"var f = function() {};",
"function (): undefined");
}
public void testFunctionInference10() throws Exception {
testFunctionType(
"/** @this Date\n@param {boolean} b\n@return {string} */" +
"var f = function(a,b) {};",
"function (this:Date, ?, boolean): string");
}
public void testFunctionInference11() throws Exception {
testFunctionType(
"var goog = {};" +
"/** @return {number}*/goog.f = function(){};",
"goog.f",
"function (): number");
}
public void testFunctionInference12() throws Exception {
testFunctionType(
"var goog = {};" +
"goog.f = function(){};",
"goog.f",
"function (): undefined");
}
public void testFunctionInference13() throws Exception {
testFunctionType(
"var goog = {};" +
"/** @constructor */ goog.Foo = function(){};" +
"/** @param {!goog.Foo} f */function eatFoo(f){};",
"eatFoo",
"function (goog.Foo): undefined");
}
public void testFunctionInference14() throws Exception {
testFunctionType(
"var goog = {};" +
"/** @constructor */ goog.Foo = function(){};" +
"/** @return {!goog.Foo} */function eatFoo(){ return new goog.Foo; };",
"eatFoo",
"function (): goog.Foo");
}
public void testFunctionInference15() throws Exception {
testFunctionType(
"/** @constructor */ function f() {};" +
"f.prototype.foo = function(){};",
"f.prototype.foo",
"function (this:f): undefined");
}
public void testFunctionInference16() throws Exception {
testFunctionType(
"/** @constructor */ function f() {};" +
"f.prototype.foo = function(){};",
"(new f).foo",
"function (this:f): undefined");
}
public void testFunctionInference17() throws Exception {
testFunctionType(
"/** @constructor */ function f() {}" +
"function abstractMethod() {}" +
"/** @param {number} x */ f.prototype.foo = abstractMethod;",
"(new f).foo",
"function (this:f, number): ?");
}
public void testFunctionInference18() throws Exception {
testFunctionType(
"var goog = {};" +
"/** @this {Date} */ goog.eatWithDate;",
"goog.eatWithDate",
"function (this:Date): ?");
}
public void testFunctionInference19() throws Exception {
testFunctionType(
"/** @param {string} x */ var f;",
"f",
"function (string): ?");
}
public void testFunctionInference20() throws Exception {
testFunctionType(
"/** @this {Date} */ var f;",
"f",
"function (this:Date): ?");
}
public void testFunctionInference21() throws Exception {
testTypes(
"var f = function() { throw 'x' };" +
"/** @return {boolean} */ var g = f;");
testFunctionType(
"var f = function() { throw 'x' };",
"f",
"function (): ?");
}
public void testFunctionInference22() throws Exception {
testTypes(
"/** @type {!Function} */ var f = function() { g(this); };" +
"/** @param {boolean} x */ var g = function(x) {};");
}
public void testFunctionInference23() throws Exception {
// We want to make sure that 'prop' isn't declared on all objects.
testTypes(
"/** @type {!Function} */ var f = function() {\n" +
" /** @type {number} */ this.prop = 3;\n" +
"};" +
"/**\n" +
" * @param {Object} x\n" +
" * @return {string}\n" +
" */ var g = function(x) { return x.prop; };");
}
public void testInnerFunction1() throws Exception {
testTypes(
"function f() {" +
" /** @type {number} */ var x = 3;\n" +
" function g() { x = null; }" +
" return x;" +
"}",
"assignment\n" +
"found : null\n" +
"required: number");
}
public void testInnerFunction2() throws Exception {
testTypes(
"/** @return {number} */\n" +
"function f() {" +
" var x = null;\n" +
" function g() { x = 3; }" +
" g();" +
" return x;" +
"}",
"inconsistent return type\n" +
"found : (null|number)\n" +
"required: number");
}
public void testInnerFunction3() throws Exception {
testTypes(
"var x = null;" +
"/** @return {number} */\n" +
"function f() {" +
" x = 3;\n" +
" /** @return {number} */\n" +
" function g() { x = true; return x; }" +
" return x;" +
"}",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testInnerFunction4() throws Exception {
testTypes(
"var x = null;" +
"/** @return {number} */\n" +
"function f() {" +
" x = '3';\n" +
" /** @return {number} */\n" +
" function g() { x = 3; return x; }" +
" return x;" +
"}",
"inconsistent return type\n" +
"found : string\n" +
"required: number");
}
public void testInnerFunction5() throws Exception {
testTypes(
"/** @return {number} */\n" +
"function f() {" +
" var x = 3;\n" +
" /** @return {number} */" +
" function g() { var x = 3;x = true; return x; }" +
" return x;" +
"}",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testInnerFunction6() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"function f() {" +
" var x = 0 || function() {};\n" +
" function g() { if (goog.isFunction(x)) { x(1); } }" +
" g();" +
"}",
"Function x: called with 1 argument(s). " +
"Function requires at least 0 argument(s) " +
"and no more than 0 argument(s).");
}
public void testInnerFunction7() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"function f() {" +
" /** @type {number|function()} */" +
" var x = 0 || function() {};\n" +
" function g() { if (goog.isFunction(x)) { x(1); } }" +
" g();" +
"}",
"Function x: called with 1 argument(s). " +
"Function requires at least 0 argument(s) " +
"and no more than 0 argument(s).");
}
public void testInnerFunction8() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"function f() {" +
" function x() {};\n" +
" function g() { if (goog.isFunction(x)) { x(1); } }" +
" g();" +
"}",
"Function x: called with 1 argument(s). " +
"Function requires at least 0 argument(s) " +
"and no more than 0 argument(s).");
}
public void testInnerFunction9() throws Exception {
testTypes(
"function f() {" +
" var x = 3;\n" +
" function g() { x = null; };\n" +
" function h() { return x == null; }" +
" return h();" +
"}");
}
public void testInnerFunction10() throws Exception {
testTypes(
"function f() {" +
" /** @type {?number} */ var x = null;" +
" /** @return {string} */" +
" function g() {" +
" if (!x) {" +
" x = 1;" +
" }" +
" return x;" +
" }" +
"}",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testInnerFunction11() throws Exception {
// TODO(nicksantos): This is actually bad inference, because
// h sets x to null. We should fix this, but for now we do it
// this way so that we don't break existing binaries. We will
// need to change TypeInference#isUnflowable to fix this.
testTypes(
"function f() {" +
" /** @type {?number} */ var x = null;" +
" /** @return {number} */" +
" function g() {" +
" x = 1;" +
" h();" +
" return x;" +
" }" +
" function h() {" +
" x = null;" +
" }" +
"}");
}
public void testAbstractMethodHandling1() throws Exception {
testTypes(
"/** @type {Function} */ var abstractFn = function() {};" +
"abstractFn(1);");
}
public void testAbstractMethodHandling2() throws Exception {
testTypes(
"var abstractFn = function() {};" +
"abstractFn(1);",
"Function abstractFn: called with 1 argument(s). " +
"Function requires at least 0 argument(s) " +
"and no more than 0 argument(s).");
}
public void testAbstractMethodHandling3() throws Exception {
testTypes(
"var goog = {};" +
"/** @type {Function} */ goog.abstractFn = function() {};" +
"goog.abstractFn(1);");
}
public void testAbstractMethodHandling4() throws Exception {
testTypes(
"var goog = {};" +
"goog.abstractFn = function() {};" +
"goog.abstractFn(1);",
"Function goog.abstractFn: called with 1 argument(s). " +
"Function requires at least 0 argument(s) " +
"and no more than 0 argument(s).");
}
public void testAbstractMethodHandling5() throws Exception {
testTypes(
"/** @type {!Function} */ var abstractFn = function() {};" +
"/** @param {number} x */ var f = abstractFn;" +
"f('x');",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testAbstractMethodHandling6() throws Exception {
testTypes(
"var goog = {};" +
"/** @type {Function} */ goog.abstractFn = function() {};" +
"/** @param {number} x */ goog.f = abstractFn;" +
"goog.f('x');",
"actual parameter 1 of goog.f does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testMethodInference1() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** @return {number} */ F.prototype.foo = function() { return 3; };" +
"/** @constructor \n * @extends {F} */ " +
"function G() {}" +
"/** @override */ G.prototype.foo = function() { return true; };",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testMethodInference2() throws Exception {
testTypes(
"var goog = {};" +
"/** @constructor */ goog.F = function() {};" +
"/** @return {number} */ goog.F.prototype.foo = " +
" function() { return 3; };" +
"/** @constructor \n * @extends {goog.F} */ " +
"goog.G = function() {};" +
"/** @override */ goog.G.prototype.foo = function() { return true; };",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testMethodInference3() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** @param {boolean} x \n * @return {number} */ " +
"F.prototype.foo = function(x) { return 3; };" +
"/** @constructor \n * @extends {F} */ " +
"function G() {}" +
"/** @override */ " +
"G.prototype.foo = function(x) { return x; };",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testMethodInference4() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** @param {boolean} x \n * @return {number} */ " +
"F.prototype.foo = function(x) { return 3; };" +
"/** @constructor \n * @extends {F} */ " +
"function G() {}" +
"/** @override */ " +
"G.prototype.foo = function(y) { return y; };",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testMethodInference5() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** @param {number} x \n * @return {string} */ " +
"F.prototype.foo = function(x) { return 'x'; };" +
"/** @constructor \n * @extends {F} */ " +
"function G() {}" +
"/** @type {number} */ G.prototype.num = 3;" +
"/** @override */ " +
"G.prototype.foo = function(y) { return this.num + y; };",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testMethodInference6() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** @param {number} x */ F.prototype.foo = function(x) { };" +
"/** @constructor \n * @extends {F} */ " +
"function G() {}" +
"/** @override */ G.prototype.foo = function() { };" +
"(new G()).foo(1);");
}
public void testMethodInference7() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"F.prototype.foo = function() { };" +
"/** @constructor \n * @extends {F} */ " +
"function G() {}" +
"/** @override */ G.prototype.foo = function(x, y) { };",
"mismatch of the foo property type and the type of the property " +
"it overrides from superclass F\n" +
"original: function (this:F): undefined\n" +
"override: function (this:G, ?, ?): undefined");
}
public void testMethodInference8() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"F.prototype.foo = function() { };" +
"/** @constructor \n * @extends {F} */ " +
"function G() {}" +
"/** @override */ " +
"G.prototype.foo = function(opt_b, var_args) { };" +
"(new G()).foo(1, 2, 3);");
}
public void testMethodInference9() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"F.prototype.foo = function() { };" +
"/** @constructor \n * @extends {F} */ " +
"function G() {}" +
"/** @override */ " +
"G.prototype.foo = function(var_args, opt_b) { };",
"variable length argument must be last");
}
public void testStaticMethodDeclaration1() throws Exception {
testTypes(
"/** @constructor */ function F() { F.foo(true); }" +
"/** @param {number} x */ F.foo = function(x) {};",
"actual parameter 1 of F.foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testStaticMethodDeclaration2() throws Exception {
testTypes(
"var goog = goog || {}; function f() { goog.foo(true); }" +
"/** @param {number} x */ goog.foo = function(x) {};",
"actual parameter 1 of goog.foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testStaticMethodDeclaration3() throws Exception {
testTypes(
"var goog = goog || {}; function f() { goog.foo(true); }" +
"goog.foo = function() {};",
"Function goog.foo: called with 1 argument(s). Function requires " +
"at least 0 argument(s) and no more than 0 argument(s).");
}
public void testDuplicateStaticMethodDecl1() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @param {number} x */ goog.foo = function(x) {};" +
"/** @param {number} x */ goog.foo = function(x) {};",
"variable goog.foo redefined with type function (number): undefined, " +
"original definition at [testcode]:1 " +
"with type function (number): undefined");
}
public void testDuplicateStaticMethodDecl2() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @param {number} x */ goog.foo = function(x) {};" +
"/** @param {number} x \n * @suppress {duplicate} */ " +
"goog.foo = function(x) {};");
}
public void testDuplicateStaticMethodDecl3() throws Exception {
testTypes(
"var goog = goog || {};" +
"goog.foo = function(x) {};" +
"goog.foo = function(x) {};");
}
public void testDuplicateStaticMethodDecl4() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @type {Function} */ goog.foo = function(x) {};" +
"goog.foo = function(x) {};");
}
public void testDuplicateStaticMethodDecl5() throws Exception {
testTypes(
"var goog = goog || {};" +
"goog.foo = function(x) {};" +
"/** @return {undefined} */ goog.foo = function(x) {};",
"variable goog.foo redefined with type function (?): undefined, " +
"original definition at [testcode]:1 with type " +
"function (?): undefined");
}
public void testDuplicateStaticPropertyDecl1() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @type {Foo} */ goog.foo;" +
"/** @type {Foo} */ goog.foo;" +
"/** @constructor */ function Foo() {}");
}
public void testDuplicateStaticPropertyDecl2() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @type {Foo} */ goog.foo;" +
"/** @type {Foo} \n * @suppress {duplicate} */ goog.foo;" +
"/** @constructor */ function Foo() {}");
}
public void testDuplicateStaticPropertyDecl3() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @type {!Foo} */ goog.foo;" +
"/** @type {string} */ goog.foo;" +
"/** @constructor */ function Foo() {}",
"variable goog.foo redefined with type string, " +
"original definition at [testcode]:1 with type Foo");
}
public void testDuplicateStaticPropertyDecl4() throws Exception {
testClosureTypesMultipleWarnings(
"var goog = goog || {};" +
"/** @type {!Foo} */ goog.foo;" +
"/** @type {string} */ goog.foo = 'x';" +
"/** @constructor */ function Foo() {}",
Lists.newArrayList(
"assignment to property foo of goog\n" +
"found : string\n" +
"required: Foo",
"variable goog.foo redefined with type string, " +
"original definition at [testcode]:1 with type Foo"));
}
public void testDuplicateStaticPropertyDecl5() throws Exception {
testClosureTypesMultipleWarnings(
"var goog = goog || {};" +
"/** @type {!Foo} */ goog.foo;" +
"/** @type {string}\n * @suppress {duplicate} */ goog.foo = 'x';" +
"/** @constructor */ function Foo() {}",
Lists.newArrayList(
"assignment to property foo of goog\n" +
"found : string\n" +
"required: Foo",
"variable goog.foo redefined with type string, " +
"original definition at [testcode]:1 with type Foo"));
}
public void testDuplicateStaticPropertyDecl6() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @type {string} */ goog.foo = 'y';" +
"/** @type {string}\n * @suppress {duplicate} */ goog.foo = 'x';");
}
public void testDuplicateStaticPropertyDecl7() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @param {string} x */ goog.foo;" +
"/** @type {function(string)} */ goog.foo;");
}
public void testDuplicateStaticPropertyDecl8() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @return {EventCopy} */ goog.foo;" +
"/** @constructor */ function EventCopy() {}" +
"/** @return {EventCopy} */ goog.foo;");
}
public void testDuplicateStaticPropertyDecl9() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @return {EventCopy} */ goog.foo;" +
"/** @return {EventCopy} */ goog.foo;" +
"/** @constructor */ function EventCopy() {}");
}
public void testDuplicateStaticPropertyDec20() throws Exception {
testTypes(
"/**\n" +
" * @fileoverview\n" +
" * @suppress {duplicate}\n" +
" */" +
"var goog = goog || {};" +
"/** @type {string} */ goog.foo = 'y';" +
"/** @type {string} */ goog.foo = 'x';");
}
public void testDuplicateLocalVarDecl() throws Exception {
testClosureTypesMultipleWarnings(
"/** @param {number} x */\n" +
"function f(x) { /** @type {string} */ var x = ''; }",
Lists.newArrayList(
"variable x redefined with type string, original definition" +
" at [testcode]:2 with type number",
"initializing variable\n" +
"found : string\n" +
"required: number"));
}
public void testDuplicateInstanceMethod1() throws Exception {
// If there's no jsdoc on the methods, then we treat them like
// any other inferred properties.
testTypes(
"/** @constructor */ function F() {}" +
"F.prototype.bar = function() {};" +
"F.prototype.bar = function() {};");
}
public void testDuplicateInstanceMethod2() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** jsdoc */ F.prototype.bar = function() {};" +
"/** jsdoc */ F.prototype.bar = function() {};",
"variable F.prototype.bar redefined with type " +
"function (this:F): undefined, original definition at " +
"[testcode]:1 with type function (this:F): undefined");
}
public void testDuplicateInstanceMethod3() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"F.prototype.bar = function() {};" +
"/** jsdoc */ F.prototype.bar = function() {};",
"variable F.prototype.bar redefined with type " +
"function (this:F): undefined, original definition at " +
"[testcode]:1 with type function (this:F): undefined");
}
public void testDuplicateInstanceMethod4() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** jsdoc */ F.prototype.bar = function() {};" +
"F.prototype.bar = function() {};");
}
public void testDuplicateInstanceMethod5() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** jsdoc \n * @return {number} */ F.prototype.bar = function() {" +
" return 3;" +
"};" +
"/** jsdoc \n * @suppress {duplicate} */ " +
"F.prototype.bar = function() { return ''; };",
"inconsistent return type\n" +
"found : string\n" +
"required: number");
}
public void testDuplicateInstanceMethod6() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** jsdoc \n * @return {number} */ F.prototype.bar = function() {" +
" return 3;" +
"};" +
"/** jsdoc \n * @return {string} * \n @suppress {duplicate} */ " +
"F.prototype.bar = function() { return ''; };",
"assignment to property bar of F.prototype\n" +
"found : function (this:F): string\n" +
"required: function (this:F): number");
}
public void testStubFunctionDeclaration1() throws Exception {
testFunctionType(
"/** @constructor */ function f() {};" +
"/** @param {number} x \n * @param {string} y \n" +
" * @return {number} */ f.prototype.foo;",
"(new f).foo",
"function (this:f, number, string): number");
}
public void testStubFunctionDeclaration2() throws Exception {
testExternFunctionType(
// externs
"/** @constructor */ function f() {};" +
"/** @constructor \n * @extends {f} */ f.subclass;",
"f.subclass",
"function (new:f.subclass): ?");
}
public void testStubFunctionDeclaration3() throws Exception {
testFunctionType(
"/** @constructor */ function f() {};" +
"/** @return {undefined} */ f.foo;",
"f.foo",
"function (): undefined");
}
public void testStubFunctionDeclaration4() throws Exception {
testFunctionType(
"/** @constructor */ function f() { " +
" /** @return {number} */ this.foo;" +
"}",
"(new f).foo",
"function (this:f): number");
}
public void testStubFunctionDeclaration5() throws Exception {
testFunctionType(
"/** @constructor */ function f() { " +
" /** @type {Function} */ this.foo;" +
"}",
"(new f).foo",
createNullableType(U2U_CONSTRUCTOR_TYPE).toString());
}
public void testStubFunctionDeclaration6() throws Exception {
testFunctionType(
"/** @constructor */ function f() {} " +
"/** @type {Function} */ f.prototype.foo;",
"(new f).foo",
createNullableType(U2U_CONSTRUCTOR_TYPE).toString());
}
public void testStubFunctionDeclaration7() throws Exception {
testFunctionType(
"/** @constructor */ function f() {} " +
"/** @type {Function} */ f.prototype.foo = function() {};",
"(new f).foo",
createNullableType(U2U_CONSTRUCTOR_TYPE).toString());
}
public void testStubFunctionDeclaration8() throws Exception {
testFunctionType(
"/** @type {Function} */ var f = function() {}; ",
"f",
createNullableType(U2U_CONSTRUCTOR_TYPE).toString());
}
public void testStubFunctionDeclaration9() throws Exception {
testFunctionType(
"/** @type {function():number} */ var f; ",
"f",
"function (): number");
}
public void testStubFunctionDeclaration10() throws Exception {
testFunctionType(
"/** @type {function(number):number} */ var f = function(x) {};",
"f",
"function (number): number");
}
public void testNestedFunctionInference1() throws Exception {
String nestedAssignOfFooAndBar =
"/** @constructor */ function f() {};" +
"f.prototype.foo = f.prototype.bar = function(){};";
testFunctionType(nestedAssignOfFooAndBar, "(new f).bar",
"function (this:f): undefined");
}
/**
* Tests the type of a function definition. The function defined by
* {@code functionDef} should be named {@code "f"}.
*/
private void testFunctionType(String functionDef, String functionType)
throws Exception {
testFunctionType(functionDef, "f", functionType);
}
/**
* Tests the type of a function definition. The function defined by
* {@code functionDef} should be named {@code functionName}.
*/
private void testFunctionType(String functionDef, String functionName,
String functionType) throws Exception {
// using the variable initialization check to verify the function's type
testTypes(
functionDef +
"/** @type number */var a=" + functionName + ";",
"initializing variable\n" +
"found : " + functionType + "\n" +
"required: number");
}
/**
* Tests the type of a function definition in externs.
* The function defined by {@code functionDef} should be
* named {@code functionName}.
*/
private void testExternFunctionType(String functionDef, String functionName,
String functionType) throws Exception {
testTypes(
functionDef,
"/** @type number */var a=" + functionName + ";",
"initializing variable\n" +
"found : " + functionType + "\n" +
"required: number", false);
}
public void testTypeRedefinition() throws Exception {
testClosureTypesMultipleWarnings("a={};/**@enum {string}*/ a.A = {ZOR:'b'};"
+ "/** @constructor */ a.A = function() {}",
Lists.newArrayList(
"variable a.A redefined with type function (new:a.A): undefined, " +
"original definition at [testcode]:1 with type enum{a.A}",
"assignment to property A of a\n" +
"found : function (new:a.A): undefined\n" +
"required: enum{a.A}"));
}
public void testIn1() throws Exception {
testTypes("'foo' in Object");
}
public void testIn2() throws Exception {
testTypes("3 in Object");
}
public void testIn3() throws Exception {
testTypes("undefined in Object");
}
public void testIn4() throws Exception {
testTypes("Date in Object",
"left side of 'in'\n" +
"found : function (new:Date, ?=, ?=, ?=, ?=, ?=, ?=, ?=): string\n" +
"required: string");
}
public void testIn5() throws Exception {
testTypes("'x' in null",
"'in' requires an object\n" +
"found : null\n" +
"required: Object");
}
public void testIn6() throws Exception {
testTypes(
"/** @param {number} x */" +
"function g(x) {}" +
"g(1 in {});",
"actual parameter 1 of g does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testIn7() throws Exception {
// Make sure we do inference in the 'in' expression.
testTypes(
"/**\n" +
" * @param {number} x\n" +
" * @return {number}\n" +
" */\n" +
"function g(x) { return 5; }" +
"function f() {" +
" var x = {};" +
" x.foo = '3';" +
" return g(x.foo) in {};" +
"}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testForIn1() throws Exception {
testTypes(
"/** @param {boolean} x */ function f(x) {}" +
"for (var k in {}) {" +
" f(k);" +
"}",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: boolean");
}
public void testForIn2() throws Exception {
testTypes(
"/** @param {boolean} x */ function f(x) {}" +
"/** @enum {string} */ var E = {FOO: 'bar'};" +
"/** @type {Object.<E, string>} */ var obj = {};" +
"var k = null;" +
"for (k in obj) {" +
" f(k);" +
"}",
"actual parameter 1 of f does not match formal parameter\n" +
"found : E.<string>\n" +
"required: boolean");
}
public void testForIn3() throws Exception {
testTypes(
"/** @param {boolean} x */ function f(x) {}" +
"/** @type {Object.<number>} */ var obj = {};" +
"for (var k in obj) {" +
" f(obj[k]);" +
"}",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: boolean");
}
public void testForIn4() throws Exception {
testTypes(
"/** @param {boolean} x */ function f(x) {}" +
"/** @enum {string} */ var E = {FOO: 'bar'};" +
"/** @type {Object.<E, Array>} */ var obj = {};" +
"for (var k in obj) {" +
" f(obj[k]);" +
"}",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (Array|null)\n" +
"required: boolean");
}
public void testForIn5() throws Exception {
testTypes(
"/** @param {boolean} x */ function f(x) {}" +
"/** @constructor */ var E = function(){};" +
"/** @type {Object.<E, number>} */ var obj = {};" +
"for (var k in obj) {" +
" f(k);" +
"}",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: boolean");
}
// TODO(nicksantos): change this to something that makes sense.
// public void testComparison1() throws Exception {
// testTypes("/**@type null */var a;" +
// "/**@type !Date */var b;" +
// "if (a==b) {}",
// "condition always evaluates to false\n" +
// "left : null\n" +
// "right: Date");
// }
public void testComparison2() throws Exception {
testTypes("/**@type number*/var a;" +
"/**@type !Date */var b;" +
"if (a!==b) {}",
"condition always evaluates to true\n" +
"left : number\n" +
"right: Date");
}
public void testComparison3() throws Exception {
// Since null == undefined in JavaScript, this code is reasonable.
testTypes("/** @type {(Object,undefined)} */var a;" +
"var b = a == null");
}
public void testComparison4() throws Exception {
testTypes("/** @type {(!Object,undefined)} */var a;" +
"/** @type {!Object} */var b;" +
"var c = a == b");
}
public void testComparison5() throws Exception {
testTypes("/** @type null */var a;" +
"/** @type null */var b;" +
"a == b",
"condition always evaluates to true\n" +
"left : null\n" +
"right: null");
}
public void testComparison6() throws Exception {
testTypes("/** @type null */var a;" +
"/** @type null */var b;" +
"a != b",
"condition always evaluates to false\n" +
"left : null\n" +
"right: null");
}
public void testComparison7() throws Exception {
testTypes("var a;" +
"var b;" +
"a == b",
"condition always evaluates to true\n" +
"left : undefined\n" +
"right: undefined");
}
public void testComparison8() throws Exception {
testTypes("/** @type {Array.<string>} */ var a = [];" +
"a[0] == null || a[1] == undefined");
}
public void testComparison9() throws Exception {
testTypes("/** @type {Array.<undefined>} */ var a = [];" +
"a[0] == null",
"condition always evaluates to true\n" +
"left : undefined\n" +
"right: null");
}
public void testComparison10() throws Exception {
testTypes("/** @type {Array.<undefined>} */ var a = [];" +
"a[0] === null");
}
public void testComparison11() throws Exception {
testTypes(
"(function(){}) == 'x'",
"condition always evaluates to false\n" +
"left : function (): undefined\n" +
"right: string");
}
public void testComparison12() throws Exception {
testTypes(
"(function(){}) == 3",
"condition always evaluates to false\n" +
"left : function (): undefined\n" +
"right: number");
}
public void testComparison13() throws Exception {
testTypes(
"(function(){}) == false",
"condition always evaluates to false\n" +
"left : function (): undefined\n" +
"right: boolean");
}
public void testComparison14() throws Exception {
testTypes("/** @type {function((Array|string), Object): number} */" +
"function f(x, y) { return x === y; }",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testComparison15() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @constructor */ function F() {}" +
"/**\n" +
" * @param {number} x\n" +
" * @constructor\n" +
" * @extends {F}\n" +
" */\n" +
"function G(x) {}\n" +
"goog.inherits(G, F);\n" +
"/**\n" +
" * @param {number} x\n" +
" * @constructor\n" +
" * @extends {G}\n" +
" */\n" +
"function H(x) {}\n" +
"goog.inherits(H, G);\n" +
"/** @param {G} x */" +
"function f(x) { return x.constructor === H; }",
null);
}
public void testDeleteOperator1() throws Exception {
testTypes(
"var x = {};" +
"/** @return {string} */ function f() { return delete x['a']; }",
"inconsistent return type\n" +
"found : boolean\n" +
"required: string");
}
public void testDeleteOperator2() throws Exception {
testTypes(
"var obj = {};" +
"/** \n" +
" * @param {string} x\n" +
" * @return {Object} */ function f(x) { return obj; }" +
"/** @param {?number} x */ function g(x) {" +
" if (x) { delete f(x)['a']; }" +
"}",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testEnumStaticMethod1() throws Exception {
testTypes(
"/** @enum */ var Foo = {AAA: 1};" +
"/** @param {number} x */ Foo.method = function(x) {};" +
"Foo.method(true);",
"actual parameter 1 of Foo.method does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testEnumStaticMethod2() throws Exception {
testTypes(
"/** @enum */ var Foo = {AAA: 1};" +
"/** @param {number} x */ Foo.method = function(x) {};" +
"function f() { Foo.method(true); }",
"actual parameter 1 of Foo.method does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testEnum1() throws Exception {
testTypes("/**@enum*/var a={BB:1,CC:2};\n" +
"/**@type {a}*/var d;d=a.BB;");
}
public void testEnum2() throws Exception {
testTypes("/**@enum*/var a={b:1}",
"enum key b must be a syntactic constant");
}
public void testEnum3() throws Exception {
testTypes("/**@enum*/var a={BB:1,BB:2}",
"variable a.BB redefined with type a.<number>, " +
"original definition at [testcode]:1 with type a.<number>");
}
public void testEnum4() throws Exception {
testTypes("/**@enum*/var a={BB:'string'}",
"assignment to property BB of enum{a}\n" +
"found : string\n" +
"required: number");
}
public void testEnum5() throws Exception {
testTypes("/**@enum {String}*/var a={BB:'string'}",
"assignment to property BB of enum{a}\n" +
"found : string\n" +
"required: (String|null)");
}
public void testEnum6() throws Exception {
testTypes("/**@enum*/var a={BB:1,CC:2};\n/**@type {!Array}*/var d;d=a.BB;",
"assignment\n" +
"found : a.<number>\n" +
"required: Array");
}
public void testEnum7() throws Exception {
testTypes("/** @enum */var a={AA:1,BB:2,CC:3};" +
"/** @type a */var b=a.D;",
"element D does not exist on this enum");
}
public void testEnum8() throws Exception {
testClosureTypesMultipleWarnings("/** @enum */var a=8;",
Lists.newArrayList(
"enum initializer must be an object literal or an enum",
"initializing variable\n" +
"found : number\n" +
"required: enum{a}"));
}
public void testEnum9() throws Exception {
testClosureTypesMultipleWarnings(
"var goog = {};" +
"/** @enum */goog.a=8;",
Lists.newArrayList(
"assignment to property a of goog\n" +
"found : number\n" +
"required: enum{goog.a}",
"enum initializer must be an object literal or an enum"));
}
public void testEnum10() throws Exception {
testTypes(
"/** @enum {number} */" +
"goog.K = { A : 3 };");
}
public void testEnum11() throws Exception {
testTypes(
"/** @enum {number} */" +
"goog.K = { 502 : 3 };");
}
public void testEnum12() throws Exception {
testTypes(
"/** @enum {number} */ var a = {};" +
"/** @enum */ var b = a;");
}
public void testEnum13() throws Exception {
testTypes(
"/** @enum {number} */ var a = {};" +
"/** @enum {string} */ var b = a;",
"incompatible enum element types\n" +
"found : number\n" +
"required: string");
}
public void testEnum14() throws Exception {
testTypes(
"/** @enum {number} */ var a = {FOO:5};" +
"/** @enum */ var b = a;" +
"var c = b.FOO;");
}
public void testEnum15() throws Exception {
testTypes(
"/** @enum {number} */ var a = {FOO:5};" +
"/** @enum */ var b = a;" +
"var c = b.BAR;",
"element BAR does not exist on this enum");
}
public void testEnum16() throws Exception {
testTypes("var goog = {};" +
"/**@enum*/goog .a={BB:1,BB:2}",
"variable goog.a.BB redefined with type goog.a.<number>, " +
"original definition at [testcode]:1 with type goog.a.<number>");
}
public void testEnum17() throws Exception {
testTypes("var goog = {};" +
"/**@enum*/goog.a={BB:'string'}",
"assignment to property BB of enum{goog.a}\n" +
"found : string\n" +
"required: number");
}
public void testEnum18() throws Exception {
testTypes("/**@enum*/ var E = {A: 1, B: 2};" +
"/** @param {!E} x\n@return {number} */\n" +
"var f = function(x) { return x; };");
}
public void testEnum19() throws Exception {
testTypes("/**@enum*/ var E = {A: 1, B: 2};" +
"/** @param {number} x\n@return {!E} */\n" +
"var f = function(x) { return x; };",
"inconsistent return type\n" +
"found : number\n" +
"required: E.<number>");
}
public void testEnum20() throws Exception {
testTypes("/**@enum*/ var E = {A: 1, B: 2}; var x = []; x[E.A] = 0;");
}
public void testEnum21() throws Exception {
Node n = parseAndTypeCheck(
"/** @enum {string} */ var E = {A : 'a', B : 'b'};\n" +
"/** @param {!E} x\n@return {!E} */ function f(x) { return x; }");
Node nodeX = n.getLastChild().getLastChild().getLastChild().getLastChild();
JSType typeE = nodeX.getJSType();
assertFalse(typeE.isObject());
assertFalse(typeE.isNullable());
}
public void testEnum22() throws Exception {
testTypes("/**@enum*/ var E = {A: 1, B: 2};" +
"/** @param {E} x \n* @return {number} */ function f(x) {return x}");
}
public void testEnum23() throws Exception {
testTypes("/**@enum*/ var E = {A: 1, B: 2};" +
"/** @param {E} x \n* @return {string} */ function f(x) {return x}",
"inconsistent return type\n" +
"found : E.<number>\n" +
"required: string");
}
public void testEnum24() throws Exception {
testTypes("/**@enum {Object} */ var E = {A: {}};" +
"/** @param {E} x \n* @return {!Object} */ function f(x) {return x}",
"inconsistent return type\n" +
"found : E.<(Object|null)>\n" +
"required: Object");
}
public void testEnum25() throws Exception {
testTypes("/**@enum {!Object} */ var E = {A: {}};" +
"/** @param {E} x \n* @return {!Object} */ function f(x) {return x}");
}
public void testEnum26() throws Exception {
testTypes("var a = {}; /**@enum*/ a.B = {A: 1, B: 2};" +
"/** @param {a.B} x \n* @return {number} */ function f(x) {return x}");
}
public void testEnum27() throws Exception {
// x is unknown
testTypes("/** @enum */ var A = {B: 1, C: 2}; " +
"function f(x) { return A == x; }");
}
public void testEnum28() throws Exception {
// x is unknown
testTypes("/** @enum */ var A = {B: 1, C: 2}; " +
"function f(x) { return A.B == x; }");
}
public void testEnum29() throws Exception {
testTypes("/** @enum */ var A = {B: 1, C: 2}; " +
"/** @return {number} */ function f() { return A; }",
"inconsistent return type\n" +
"found : enum{A}\n" +
"required: number");
}
public void testEnum30() throws Exception {
testTypes("/** @enum */ var A = {B: 1, C: 2}; " +
"/** @return {number} */ function f() { return A.B; }");
}
public void testEnum31() throws Exception {
testTypes("/** @enum */ var A = {B: 1, C: 2}; " +
"/** @return {A} */ function f() { return A; }",
"inconsistent return type\n" +
"found : enum{A}\n" +
"required: A.<number>");
}
public void testEnum32() throws Exception {
testTypes("/** @enum */ var A = {B: 1, C: 2}; " +
"/** @return {A} */ function f() { return A.B; }");
}
public void testEnum34() throws Exception {
testTypes("/** @enum */ var A = {B: 1, C: 2}; " +
"/** @param {number} x */ function f(x) { return x == A.B; }");
}
public void testEnum35() throws Exception {
testTypes("var a = a || {}; /** @enum */ a.b = {C: 1, D: 2};" +
"/** @return {a.b} */ function f() { return a.b.C; }");
}
public void testEnum36() throws Exception {
testTypes("var a = a || {}; /** @enum */ a.b = {C: 1, D: 2};" +
"/** @return {!a.b} */ function f() { return 1; }",
"inconsistent return type\n" +
"found : number\n" +
"required: a.b.<number>");
}
public void testEnum37() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @enum {number} */ goog.a = {};" +
"/** @enum */ var b = goog.a;");
}
public void testEnum38() throws Exception {
testTypes(
"/** @enum {MyEnum} */ var MyEnum = {};" +
"/** @param {MyEnum} x */ function f(x) {}",
"Parse error. Cycle detected in inheritance chain " +
"of type MyEnum");
}
public void testEnum39() throws Exception {
testTypes(
"/** @enum {Number} */ var MyEnum = {FOO: new Number(1)};" +
"/** @param {MyEnum} x \n * @return {number} */" +
"function f(x) { return x == MyEnum.FOO && MyEnum.FOO == x; }",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testEnum40() throws Exception {
testTypes(
"/** @enum {Number} */ var MyEnum = {FOO: new Number(1)};" +
"/** @param {number} x \n * @return {number} */" +
"function f(x) { return x == MyEnum.FOO && MyEnum.FOO == x; }",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testEnum41() throws Exception {
testTypes(
"/** @enum {number} */ var MyEnum = {/** @const */ FOO: 1};" +
"/** @return {string} */" +
"function f() { return MyEnum.FOO; }",
"inconsistent return type\n" +
"found : MyEnum.<number>\n" +
"required: string");
}
public void testEnum42() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"/** @enum {Object} */ var MyEnum = {FOO: {newProperty: 1, b: 2}};" +
"f(MyEnum.FOO.newProperty);");
}
public void testAliasedEnum1() throws Exception {
testTypes(
"/** @enum */ var YourEnum = {FOO: 3};" +
"/** @enum */ var MyEnum = YourEnum;" +
"/** @param {MyEnum} x */ function f(x) {} f(MyEnum.FOO);");
}
public void testAliasedEnum2() throws Exception {
testTypes(
"/** @enum */ var YourEnum = {FOO: 3};" +
"/** @enum */ var MyEnum = YourEnum;" +
"/** @param {YourEnum} x */ function f(x) {} f(MyEnum.FOO);");
}
public void testAliasedEnum3() throws Exception {
testTypes(
"/** @enum */ var YourEnum = {FOO: 3};" +
"/** @enum */ var MyEnum = YourEnum;" +
"/** @param {MyEnum} x */ function f(x) {} f(YourEnum.FOO);");
}
public void testAliasedEnum4() throws Exception {
testTypes(
"/** @enum */ var YourEnum = {FOO: 3};" +
"/** @enum */ var MyEnum = YourEnum;" +
"/** @param {YourEnum} x */ function f(x) {} f(YourEnum.FOO);");
}
public void testAliasedEnum5() throws Exception {
testTypes(
"/** @enum */ var YourEnum = {FOO: 3};" +
"/** @enum */ var MyEnum = YourEnum;" +
"/** @param {string} x */ function f(x) {} f(MyEnum.FOO);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : YourEnum.<number>\n" +
"required: string");
}
public void testBackwardsEnumUse1() throws Exception {
testTypes(
"/** @return {string} */ function f() { return MyEnum.FOO; }" +
"/** @enum {string} */ var MyEnum = {FOO: 'x'};");
}
public void testBackwardsEnumUse2() throws Exception {
testTypes(
"/** @return {number} */ function f() { return MyEnum.FOO; }" +
"/** @enum {string} */ var MyEnum = {FOO: 'x'};",
"inconsistent return type\n" +
"found : MyEnum.<string>\n" +
"required: number");
}
public void testBackwardsEnumUse3() throws Exception {
testTypes(
"/** @return {string} */ function f() { return MyEnum.FOO; }" +
"/** @enum {string} */ var YourEnum = {FOO: 'x'};" +
"/** @enum {string} */ var MyEnum = YourEnum;");
}
public void testBackwardsEnumUse4() throws Exception {
testTypes(
"/** @return {number} */ function f() { return MyEnum.FOO; }" +
"/** @enum {string} */ var YourEnum = {FOO: 'x'};" +
"/** @enum {string} */ var MyEnum = YourEnum;",
"inconsistent return type\n" +
"found : YourEnum.<string>\n" +
"required: number");
}
public void testBackwardsEnumUse5() throws Exception {
testTypes(
"/** @return {string} */ function f() { return MyEnum.BAR; }" +
"/** @enum {string} */ var YourEnum = {FOO: 'x'};" +
"/** @enum {string} */ var MyEnum = YourEnum;",
"element BAR does not exist on this enum");
}
public void testBackwardsTypedefUse2() throws Exception {
testTypes(
"/** @this {MyTypedef} */ function f() {}" +
"/** @typedef {!(Date|Array)} */ var MyTypedef;");
}
public void testBackwardsTypedefUse4() throws Exception {
testTypes(
"/** @return {MyTypedef} */ function f() { return null; }" +
"/** @typedef {string} */ var MyTypedef;",
"inconsistent return type\n" +
"found : null\n" +
"required: string");
}
public void testBackwardsTypedefUse6() throws Exception {
testTypes(
"/** @return {goog.MyTypedef} */ function f() { return null; }" +
"var goog = {};" +
"/** @typedef {string} */ goog.MyTypedef;",
"inconsistent return type\n" +
"found : null\n" +
"required: string");
}
public void testBackwardsTypedefUse7() throws Exception {
testTypes(
"/** @return {goog.MyTypedef} */ function f() { return null; }" +
"var goog = {};" +
"/** @typedef {Object} */ goog.MyTypedef;");
}
public void testBackwardsTypedefUse8() throws Exception {
// Technically, this isn't quite right, because the JS runtime
// will coerce null -> the global object. But we'll punt on that for now.
testTypes(
"/** @param {!Array} x */ function g(x) {}" +
"/** @this {goog.MyTypedef} */ function f() { g(this); }" +
"var goog = {};" +
"/** @typedef {(Array|null|undefined)} */ goog.MyTypedef;");
}
public void testBackwardsTypedefUse9() throws Exception {
testTypes(
"/** @param {!Array} x */ function g(x) {}" +
"/** @this {goog.MyTypedef} */ function f() { g(this); }" +
"var goog = {};" +
"/** @typedef {(Error|null|undefined)} */ goog.MyTypedef;",
"actual parameter 1 of g does not match formal parameter\n" +
"found : Error\n" +
"required: Array");
}
public void testBackwardsTypedefUse10() throws Exception {
testTypes(
"/** @param {goog.MyEnum} x */ function g(x) {}" +
"var goog = {};" +
"/** @enum {goog.MyTypedef} */ goog.MyEnum = {FOO: 1};" +
"/** @typedef {number} */ goog.MyTypedef;" +
"g(1);",
"actual parameter 1 of g does not match formal parameter\n" +
"found : number\n" +
"required: goog.MyEnum.<number>");
}
public void testBackwardsConstructor1() throws Exception {
testTypes(
"function f() { (new Foo(true)); }" +
"/** \n * @constructor \n * @param {number} x */" +
"var Foo = function(x) {};",
"actual parameter 1 of Foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testBackwardsConstructor2() throws Exception {
testTypes(
"function f() { (new Foo(true)); }" +
"/** \n * @constructor \n * @param {number} x */" +
"var YourFoo = function(x) {};" +
"/** \n * @constructor \n * @param {number} x */" +
"var Foo = YourFoo;",
"actual parameter 1 of Foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testMinimalConstructorAnnotation() throws Exception {
testTypes("/** @constructor */function Foo(){}");
}
public void testGoodExtends1() throws Exception {
// A minimal @extends example
testTypes("/** @constructor */function base() {}\n" +
"/** @constructor\n * @extends {base} */function derived() {}\n");
}
public void testGoodExtends2() throws Exception {
testTypes("/** @constructor\n * @extends base */function derived() {}\n" +
"/** @constructor */function base() {}\n");
}
public void testGoodExtends3() throws Exception {
testTypes("/** @constructor\n * @extends {Object} */function base() {}\n" +
"/** @constructor\n * @extends {base} */function derived() {}\n");
}
public void testGoodExtends4() throws Exception {
// Ensure that @extends actually sets the base type of a constructor
// correctly. Because this isn't part of the human-readable Function
// definition, we need to crawl the prototype chain (eww).
Node n = parseAndTypeCheck(
"var goog = {};\n" +
"/** @constructor */goog.Base = function(){};\n" +
"/** @constructor\n" +
" * @extends {goog.Base} */goog.Derived = function(){};\n");
Node subTypeName = n.getLastChild().getLastChild().getFirstChild();
assertEquals("goog.Derived", subTypeName.getQualifiedName());
FunctionType subCtorType =
(FunctionType) subTypeName.getNext().getJSType();
assertEquals("goog.Derived", subCtorType.getInstanceType().toString());
JSType superType = subCtorType.getPrototype().getImplicitPrototype();
assertEquals("goog.Base", superType.toString());
}
public void testGoodExtends5() throws Exception {
// we allow for the extends annotation to be placed first
testTypes("/** @constructor */function base() {}\n" +
"/** @extends {base}\n * @constructor */function derived() {}\n");
}
public void testGoodExtends6() throws Exception {
testFunctionType(
CLOSURE_DEFS +
"/** @constructor */function base() {}\n" +
"/** @return {number} */ " +
" base.prototype.foo = function() { return 1; };\n" +
"/** @extends {base}\n * @constructor */function derived() {}\n" +
"goog.inherits(derived, base);",
"derived.superClass_.foo",
"function (this:base): number");
}
public void testGoodExtends7() throws Exception {
testFunctionType(
"Function.prototype.inherits = function(x) {};" +
"/** @constructor */function base() {}\n" +
"/** @extends {base}\n * @constructor */function derived() {}\n" +
"derived.inherits(base);",
"(new derived).constructor",
"function (new:derived, ...[?]): ?");
}
public void testGoodExtends8() throws Exception {
testTypes("/** @constructor \n @extends {Base} */ function Sub() {}" +
"/** @return {number} */ function f() { return (new Sub()).foo; }" +
"/** @constructor */ function Base() {}" +
"/** @type {boolean} */ Base.prototype.foo = true;",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testGoodExtends9() throws Exception {
testTypes(
"/** @constructor */ function Super() {}" +
"Super.prototype.foo = function() {};" +
"/** @constructor \n * @extends {Super} */ function Sub() {}" +
"Sub.prototype = new Super();" +
"/** @override */ Sub.prototype.foo = function() {};");
}
public void testGoodExtends10() throws Exception {
testTypes(
"/** @constructor */ function Super() {}" +
"/** @constructor \n * @extends {Super} */ function Sub() {}" +
"Sub.prototype = new Super();" +
"/** @return {Super} */ function foo() { return new Sub(); }");
}
public void testGoodExtends11() throws Exception {
testTypes(
"/** @constructor */ function Super() {}" +
"/** @param {boolean} x */ Super.prototype.foo = function(x) {};" +
"/** @constructor \n * @extends {Super} */ function Sub() {}" +
"Sub.prototype = new Super();" +
"(new Sub()).foo(0);",
"actual parameter 1 of Super.prototype.foo " +
"does not match formal parameter\n" +
"found : number\n" +
"required: boolean");
}
public void testGoodExtends12() throws Exception {
testTypes(
"/** @constructor \n * @extends {Super} */ function Sub() {}" +
"/** @constructor \n * @extends {Sub} */ function Sub2() {}" +
"/** @constructor */ function Super() {}" +
"/** @param {Super} x */ function foo(x) {}" +
"foo(new Sub2());");
}
public void testGoodExtends13() throws Exception {
testTypes(
"/** @constructor \n * @extends {B} */ function C() {}" +
"/** @constructor \n * @extends {D} */ function E() {}" +
"/** @constructor \n * @extends {C} */ function D() {}" +
"/** @constructor \n * @extends {A} */ function B() {}" +
"/** @constructor */ function A() {}" +
"/** @param {number} x */ function f(x) {} f(new E());",
"actual parameter 1 of f does not match formal parameter\n" +
"found : E\n" +
"required: number");
}
public void testGoodExtends14() throws Exception {
testTypes(
CLOSURE_DEFS +
"/** @param {Function} f */ function g(f) {" +
" /** @constructor */ function NewType() {};" +
" goog.inherits(NewType, f);" +
" (new NewType());" +
"}");
}
public void testGoodExtends15() throws Exception {
testTypes(
CLOSURE_DEFS +
"/** @constructor */ function OldType() {}" +
"/** @param {?function(new:OldType)} f */ function g(f) {" +
" /**\n" +
" * @constructor\n" +
" * @extends {OldType}\n" +
" */\n" +
" function NewType() {};" +
" goog.inherits(NewType, f);" +
" NewType.prototype.method = function() {" +
" NewType.superClass_.foo.call(this);" +
" };" +
"}",
"Property foo never defined on OldType.prototype");
}
public void testGoodExtends16() throws Exception {
testTypes(
CLOSURE_DEFS +
"/** @param {Function} f */ function g(f) {" +
" /** @constructor */ function NewType() {};" +
" goog.inherits(f, NewType);" +
" (new NewType());" +
"}");
}
public void testGoodExtends17() throws Exception {
testFunctionType(
"Function.prototype.inherits = function(x) {};" +
"/** @constructor */function base() {}\n" +
"/** @param {number} x */ base.prototype.bar = function(x) {};\n" +
"/** @extends {base}\n * @constructor */function derived() {}\n" +
"derived.inherits(base);",
"(new derived).constructor.prototype.bar",
"function (this:base, number): undefined");
}
public void testBadExtends1() throws Exception {
testTypes("/** @constructor */function base() {}\n" +
"/** @constructor\n * @extends {not_base} */function derived() {}\n",
"Bad type annotation. Unknown type not_base");
}
public void testBadExtends2() throws Exception {
testTypes("/** @constructor */function base() {\n" +
"/** @type {!Number}*/\n" +
"this.baseMember = new Number(4);\n" +
"}\n" +
"/** @constructor\n" +
" * @extends {base} */function derived() {}\n" +
"/** @param {!String} x*/\n" +
"function foo(x){ }\n" +
"/** @type {!derived}*/var y;\n" +
"foo(y.baseMember);\n",
"actual parameter 1 of foo does not match formal parameter\n" +
"found : Number\n" +
"required: String");
}
public void testBadExtends3() throws Exception {
testTypes("/** @extends {Object} */function base() {}",
"@extends used without @constructor or @interface for base");
}
public void testBadExtends4() throws Exception {
// If there's a subclass of a class with a bad extends,
// we only want to warn about the first one.
testTypes(
"/** @constructor \n * @extends {bad} */ function Sub() {}" +
"/** @constructor \n * @extends {Sub} */ function Sub2() {}" +
"/** @param {Sub} x */ function foo(x) {}" +
"foo(new Sub2());",
"Bad type annotation. Unknown type bad");
}
public void testLateExtends() throws Exception {
testTypes(
CLOSURE_DEFS +
"/** @constructor */ function Foo() {}\n" +
"Foo.prototype.foo = function() {};\n" +
"/** @constructor */function Bar() {}\n" +
"goog.inherits(Foo, Bar);\n",
"Missing @extends tag on type Foo");
}
public void testSuperclassMatch() throws Exception {
compiler.getOptions().setCodingConvention(new GoogleCodingConvention());
testTypes("/** @constructor */ var Foo = function() {};\n" +
"/** @constructor \n @extends Foo */ var Bar = function() {};\n" +
"Bar.inherits = function(x){};" +
"Bar.inherits(Foo);\n");
}
public void testSuperclassMatchWithMixin() throws Exception {
compiler.getOptions().setCodingConvention(new GoogleCodingConvention());
testTypes("/** @constructor */ var Foo = function() {};\n" +
"/** @constructor */ var Baz = function() {};\n" +
"/** @constructor \n @extends Foo */ var Bar = function() {};\n" +
"Bar.inherits = function(x){};" +
"Bar.mixin = function(y){};" +
"Bar.inherits(Foo);\n" +
"Bar.mixin(Baz);\n");
}
public void testSuperclassMismatch1() throws Exception {
compiler.getOptions().setCodingConvention(new GoogleCodingConvention());
testTypes("/** @constructor */ var Foo = function() {};\n" +
"/** @constructor \n @extends Object */ var Bar = function() {};\n" +
"Bar.inherits = function(x){};" +
"Bar.inherits(Foo);\n",
"Missing @extends tag on type Bar");
}
public void testSuperclassMismatch2() throws Exception {
compiler.getOptions().setCodingConvention(new GoogleCodingConvention());
testTypes("/** @constructor */ var Foo = function(){};\n" +
"/** @constructor */ var Bar = function(){};\n" +
"Bar.inherits = function(x){};" +
"Bar.inherits(Foo);",
"Missing @extends tag on type Bar");
}
public void testSuperClassDefinedAfterSubClass1() throws Exception {
testTypes(
"/** @constructor \n * @extends {Base} */ function A() {}" +
"/** @constructor \n * @extends {Base} */ function B() {}" +
"/** @constructor */ function Base() {}" +
"/** @param {A|B} x \n * @return {B|A} */ " +
"function foo(x) { return x; }");
}
public void testSuperClassDefinedAfterSubClass2() throws Exception {
testTypes(
"/** @constructor \n * @extends {Base} */ function A() {}" +
"/** @constructor \n * @extends {Base} */ function B() {}" +
"/** @param {A|B} x \n * @return {B|A} */ " +
"function foo(x) { return x; }" +
"/** @constructor */ function Base() {}");
}
public void testDirectPrototypeAssignment1() throws Exception {
testTypes(
"/** @constructor */ function Base() {}" +
"Base.prototype.foo = 3;" +
"/** @constructor \n * @extends {Base} */ function A() {}" +
"A.prototype = new Base();" +
"/** @return {string} */ function foo() { return (new A).foo; }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testDirectPrototypeAssignment2() throws Exception {
// This ensures that we don't attach property 'foo' onto the Base
// instance object.
testTypes(
"/** @constructor */ function Base() {}" +
"/** @constructor \n * @extends {Base} */ function A() {}" +
"A.prototype = new Base();" +
"A.prototype.foo = 3;" +
"/** @return {string} */ function foo() { return (new Base).foo; }");
}
public void testDirectPrototypeAssignment3() throws Exception {
// This verifies that the compiler doesn't crash if the user
// overwrites the prototype of a global variable in a local scope.
testTypes(
"/** @constructor */ var MainWidgetCreator = function() {};" +
"/** @param {Function} ctor */" +
"function createMainWidget(ctor) {" +
" /** @constructor */ function tempCtor() {};" +
" tempCtor.prototype = ctor.prototype;" +
" MainWidgetCreator.superClass_ = ctor.prototype;" +
" MainWidgetCreator.prototype = new tempCtor();" +
"}");
}
public void testGoodImplements1() throws Exception {
testTypes("/** @interface */function Disposable() {}\n" +
"/** @implements {Disposable}\n * @constructor */function f() {}");
}
public void testGoodImplements2() throws Exception {
testTypes("/** @interface */function Base1() {}\n" +
"/** @interface */function Base2() {}\n" +
"/** @constructor\n" +
" * @implements {Base1}\n" +
" * @implements {Base2}\n" +
" */ function derived() {}");
}
public void testGoodImplements3() throws Exception {
testTypes("/** @interface */function Disposable() {}\n" +
"/** @constructor \n @implements {Disposable} */function f() {}");
}
public void testGoodImplements4() throws Exception {
testTypes("var goog = {};" +
"/** @type {!Function} */" +
"goog.abstractMethod = function() {};" +
"/** @interface */\n" +
"goog.Disposable = goog.abstractMethod;" +
"goog.Disposable.prototype.dispose = goog.abstractMethod;" +
"/** @implements {goog.Disposable}\n * @constructor */" +
"goog.SubDisposable = function() {};" +
"/** @inheritDoc */ " +
"goog.SubDisposable.prototype.dispose = function() {};");
}
public void testGoodImplements5() throws Exception {
testTypes(
"/** @interface */\n" +
"goog.Disposable = function() {};" +
"/** @type {Function} */" +
"goog.Disposable.prototype.dispose = function() {};" +
"/** @implements {goog.Disposable}\n * @constructor */" +
"goog.SubDisposable = function() {};" +
"/** @param {number} key \n @override */ " +
"goog.SubDisposable.prototype.dispose = function(key) {};");
}
public void testGoodImplements6() throws Exception {
testTypes(
"var myNullFunction = function() {};" +
"/** @interface */\n" +
"goog.Disposable = function() {};" +
"/** @return {number} */" +
"goog.Disposable.prototype.dispose = myNullFunction;" +
"/** @implements {goog.Disposable}\n * @constructor */" +
"goog.SubDisposable = function() {};" +
"/** @return {number} \n @override */ " +
"goog.SubDisposable.prototype.dispose = function() { return 0; };");
}
public void testGoodImplements7() throws Exception {
testTypes(
"var myNullFunction = function() {};" +
"/** @interface */\n" +
"goog.Disposable = function() {};" +
"/** @return {number} */" +
"goog.Disposable.prototype.dispose = function() {};" +
"/** @implements {goog.Disposable}\n * @constructor */" +
"goog.SubDisposable = function() {};" +
"/** @return {number} \n @override */ " +
"goog.SubDisposable.prototype.dispose = function() { return 0; };");
}
public void testBadImplements1() throws Exception {
testTypes("/** @interface */function Base1() {}\n" +
"/** @interface */function Base2() {}\n" +
"/** @constructor\n" +
" * @implements {nonExistent}\n" +
" * @implements {Base2}\n" +
" */ function derived() {}",
"Bad type annotation. Unknown type nonExistent");
}
public void testBadImplements2() throws Exception {
testTypes("/** @interface */function Disposable() {}\n" +
"/** @implements {Disposable}\n */function f() {}",
"@implements used without @constructor for f");
}
public void testBadImplements3() throws Exception {
testTypes(
"var goog = {};" +
"/** @type {!Function} */ goog.abstractMethod = function(){};" +
"/** @interface */ var Disposable = goog.abstractMethod;" +
"Disposable.prototype.method = goog.abstractMethod;" +
"/** @implements {Disposable}\n * @constructor */function f() {}",
"property method on interface Disposable is not implemented by type f");
}
public void testBadImplements4() throws Exception {
testTypes("/** @interface */function Disposable() {}\n" +
"/** @implements {Disposable}\n * @interface */function f() {}",
"f cannot implement this type; an interface can only extend, " +
"but not implement interfaces");
}
public void testBadImplements5() throws Exception {
testTypes("/** @interface */function Disposable() {}\n" +
"/** @type {number} */ Disposable.prototype.bar = function() {};",
"assignment to property bar of Disposable.prototype\n" +
"found : function (): undefined\n" +
"required: number");
}
public void testBadImplements6() throws Exception {
testClosureTypesMultipleWarnings(
"/** @interface */function Disposable() {}\n" +
"/** @type {function()} */ Disposable.prototype.bar = 3;",
Lists.newArrayList(
"assignment to property bar of Disposable.prototype\n" +
"found : number\n" +
"required: function (): ?",
"interface members can only be empty property declarations, " +
"empty functions, or goog.abstractMethod"));
}
public void testInterfaceExtends() throws Exception {
testTypes("/** @interface */function A() {}\n" +
"/** @interface \n * @extends {A} */function B() {}\n" +
"/** @constructor\n" +
" * @implements {B}\n" +
" */ function derived() {}");
}
public void testBadInterfaceExtends1() throws Exception {
testTypes("/** @interface \n * @extends {nonExistent} */function A() {}",
"Bad type annotation. Unknown type nonExistent");
}
public void testBadInterfaceExtends2() throws Exception {
testTypes("/** @constructor */function A() {}\n" +
"/** @interface \n * @extends {A} */function B() {}",
"B cannot extend this type; interfaces can only extend interfaces");
}
public void testBadInterfaceExtends3() throws Exception {
testTypes("/** @interface */function A() {}\n" +
"/** @constructor \n * @extends {A} */function B() {}",
"B cannot extend this type; constructors can only extend constructors");
}
public void testBadInterfaceExtends4() throws Exception {
// TODO(user): This should be detected as an error. Even if we enforce
// that A cannot be used in the assignment, we should still detect the
// inheritance chain as invalid.
testTypes("/** @interface */function A() {}\n" +
"/** @constructor */function B() {}\n" +
"B.prototype = A;");
}
public void testBadInterfaceExtends5() throws Exception {
// TODO(user): This should be detected as an error. Even if we enforce
// that A cannot be used in the assignment, we should still detect the
// inheritance chain as invalid.
testTypes("/** @constructor */function A() {}\n" +
"/** @interface */function B() {}\n" +
"B.prototype = A;");
}
public void testBadImplementsAConstructor() throws Exception {
testTypes("/** @constructor */function A() {}\n" +
"/** @constructor \n * @implements {A} */function B() {}",
"can only implement interfaces");
}
public void testBadImplementsNonInterfaceType() throws Exception {
testTypes("/** @constructor \n * @implements {Boolean} */function B() {}",
"can only implement interfaces");
}
public void testBadImplementsNonObjectType() throws Exception {
testTypes("/** @constructor \n * @implements {string} */function S() {}",
"can only implement interfaces");
}
public void testInterfaceAssignment1() throws Exception {
testTypes("/** @interface */var I = function() {};\n" +
"/** @constructor\n@implements {I} */var T = function() {};\n" +
"var t = new T();\n" +
"/** @type {!I} */var i = t;");
}
public void testInterfaceAssignment2() throws Exception {
testTypes("/** @interface */var I = function() {};\n" +
"/** @constructor */var T = function() {};\n" +
"var t = new T();\n" +
"/** @type {!I} */var i = t;",
"initializing variable\n" +
"found : T\n" +
"required: I");
}
public void testInterfaceAssignment3() throws Exception {
testTypes("/** @interface */var I = function() {};\n" +
"/** @constructor\n@implements {I} */var T = function() {};\n" +
"var t = new T();\n" +
"/** @type {I|number} */var i = t;");
}
public void testInterfaceAssignment4() throws Exception {
testTypes("/** @interface */var I1 = function() {};\n" +
"/** @interface */var I2 = function() {};\n" +
"/** @constructor\n@implements {I1} */var T = function() {};\n" +
"var t = new T();\n" +
"/** @type {I1|I2} */var i = t;");
}
public void testInterfaceAssignment5() throws Exception {
testTypes("/** @interface */var I1 = function() {};\n" +
"/** @interface */var I2 = function() {};\n" +
"/** @constructor\n@implements {I1}\n@implements {I2}*/" +
"var T = function() {};\n" +
"var t = new T();\n" +
"/** @type {I1} */var i1 = t;\n" +
"/** @type {I2} */var i2 = t;\n");
}
public void testInterfaceAssignment6() throws Exception {
testTypes("/** @interface */var I1 = function() {};\n" +
"/** @interface */var I2 = function() {};\n" +
"/** @constructor\n@implements {I1} */var T = function() {};\n" +
"/** @type {!I1} */var i1 = new T();\n" +
"/** @type {!I2} */var i2 = i1;\n",
"initializing variable\n" +
"found : I1\n" +
"required: I2");
}
public void testInterfaceAssignment7() throws Exception {
testTypes("/** @interface */var I1 = function() {};\n" +
"/** @interface\n@extends {I1}*/var I2 = function() {};\n" +
"/** @constructor\n@implements {I2}*/var T = function() {};\n" +
"var t = new T();\n" +
"/** @type {I1} */var i1 = t;\n" +
"/** @type {I2} */var i2 = t;\n" +
"i1 = i2;\n");
}
public void testInterfaceAssignment8() throws Exception {
testTypes("/** @interface */var I = function() {};\n" +
"/** @type {I} */var i;\n" +
"/** @type {Object} */var o = i;\n" +
"new Object().prototype = i.prototype;");
}
public void testInterfaceAssignment9() throws Exception {
testTypes("/** @interface */var I = function() {};\n" +
"/** @return {I?} */function f() { return null; }\n" +
"/** @type {!I} */var i = f();\n",
"initializing variable\n" +
"found : (I|null)\n" +
"required: I");
}
public void testInterfaceAssignment10() throws Exception {
testTypes("/** @interface */var I1 = function() {};\n" +
"/** @interface */var I2 = function() {};\n" +
"/** @constructor\n@implements {I2} */var T = function() {};\n" +
"/** @return {!I1|!I2} */function f() { return new T(); }\n" +
"/** @type {!I1} */var i1 = f();\n",
"initializing variable\n" +
"found : (I1|I2)\n" +
"required: I1");
}
public void testInterfaceAssignment11() throws Exception {
testTypes("/** @interface */var I1 = function() {};\n" +
"/** @interface */var I2 = function() {};\n" +
"/** @constructor */var T = function() {};\n" +
"/** @return {!I1|!I2|!T} */function f() { return new T(); }\n" +
"/** @type {!I1} */var i1 = f();\n",
"initializing variable\n" +
"found : (I1|I2|T)\n" +
"required: I1");
}
public void testInterfaceAssignment12() throws Exception {
testTypes("/** @interface */var I = function() {};\n" +
"/** @constructor\n@implements{I}*/var T1 = function() {};\n" +
"/** @constructor\n@extends {T1}*/var T2 = function() {};\n" +
"/** @return {I} */function f() { return new T2(); }");
}
public void testInterfaceAssignment13() throws Exception {
testTypes("/** @interface */var I = function() {};\n" +
"/** @constructor\n@implements {I}*/var T = function() {};\n" +
"/** @constructor */function Super() {};\n" +
"/** @return {I} */Super.prototype.foo = " +
"function() { return new T(); };\n" +
"/** @constructor\n@extends {Super} */function Sub() {}\n" +
"/** @override\n@return {T} */Sub.prototype.foo = " +
"function() { return new T(); };\n");
}
public void testGetprop1() throws Exception {
testTypes("/** @return {void}*/function foo(){foo().bar;}",
"No properties on this expression\n" +
"found : undefined\n" +
"required: Object");
}
public void testGetprop2() throws Exception {
testTypes("var x = null; x.alert();",
"No properties on this expression\n" +
"found : null\n" +
"required: Object");
}
public void testGetprop3() throws Exception {
testTypes(
"/** @constructor */ " +
"function Foo() { /** @type {?Object} */ this.x = null; }" +
"Foo.prototype.initX = function() { this.x = {foo: 1}; };" +
"Foo.prototype.bar = function() {" +
" if (this.x == null) { this.initX(); alert(this.x.foo); }" +
"};");
}
public void testGetprop4() throws Exception {
testTypes("var x = null; x.prop = 3;",
"No properties on this expression\n" +
"found : null\n" +
"required: Object");
}
public void testSetprop1() throws Exception {
// Create property on struct in the constructor
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */\n" +
"function Foo() { this.x = 123; }");
}
public void testSetprop2() throws Exception {
// Create property on struct outside the constructor
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */\n" +
"function Foo() {}\n" +
"(new Foo()).x = 123;",
"Cannot add a property to a struct instance " +
"after it is constructed.");
}
public void testSetprop3() throws Exception {
// Create property on struct outside the constructor
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */\n" +
"function Foo() {}\n" +
"(function() { (new Foo()).x = 123; })();",
"Cannot add a property to a struct instance " +
"after it is constructed.");
}
public void testSetprop4() throws Exception {
// Assign to existing property of struct outside the constructor
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */\n" +
"function Foo() { this.x = 123; }\n" +
"(new Foo()).x = \"asdf\";");
}
public void testSetprop5() throws Exception {
// Create a property on union that includes a struct
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */\n" +
"function Foo() {}\n" +
"(true ? new Foo() : {}).x = 123;",
"Cannot add a property to a struct instance " +
"after it is constructed.");
}
public void testSetprop6() throws Exception {
// Create property on struct in another constructor
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */\n" +
"function Foo() {}\n" +
"/**\n" +
" * @constructor\n" +
" * @param{Foo} f\n" +
" */\n" +
"function Bar(f) { f.x = 123; }",
"Cannot add a property to a struct instance " +
"after it is constructed.");
}
public void testSetprop7() throws Exception {
//Bug b/c we require THIS when creating properties on structs for simplicity
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */\n" +
"function Foo() {\n" +
" var t = this;\n" +
" t.x = 123;\n" +
"}",
"Cannot add a property to a struct instance " +
"after it is constructed.");
}
public void testSetprop8() throws Exception {
// Create property on struct using DEC
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */\n" +
"function Foo() {}\n" +
"(new Foo()).x--;",
new String[] {
"Property x never defined on Foo",
"Cannot add a property to a struct instance " +
"after it is constructed."
});
}
public void testSetprop9() throws Exception {
// Create property on struct using ASSIGN_ADD
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */\n" +
"function Foo() {}\n" +
"(new Foo()).x += 123;",
new String[] {
"Property x never defined on Foo",
"Cannot add a property to a struct instance " +
"after it is constructed."
});
}
public void testSetprop10() throws Exception {
// Create property on object literal that is a struct
testTypes("/** \n" +
" * @constructor \n" +
" * @struct \n" +
" */ \n" +
"function Square(side) { \n" +
" this.side = side; \n" +
"} \n" +
"Square.prototype = /** @struct */ {\n" +
" area: function() { return this.side * this.side; }\n" +
"};\n" +
"Square.prototype.id = function(x) { return x; };\n",
"Cannot add a property to a struct instance " +
"after it is constructed.");
}
public void testSetprop11() throws Exception {
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */\n" +
"function Foo() {}\n" +
"function Bar() {}\n" +
"Bar.prototype = new Foo();\n" +
"Bar.prototype.someprop = 123;\n",
"Cannot add a property to a struct instance " +
"after it is constructed.");
}
public void testGetpropDict1() throws Exception {
testTypes("/**\n" +
" * @constructor\n" +
" * @dict\n" +
" */" +
"function Dict1(){ this['prop'] = 123; }" +
"/** @param{Dict1} x */" +
"function takesDict(x) { return x.prop; }",
"Cannot do '.' access on a dict");
}
public void testGetpropDict2() throws Exception {
testTypes("/**\n" +
" * @constructor\n" +
" * @dict\n" +
" */" +
"function Dict1(){ this['prop'] = 123; }" +
"/**\n" +
" * @constructor\n" +
" * @extends {Dict1}\n" +
" */" +
"function Dict1kid(){ this['prop'] = 123; }" +
"/** @param{Dict1kid} x */" +
"function takesDict(x) { return x.prop; }",
"Cannot do '.' access on a dict");
}
public void testGetpropDict3() throws Exception {
testTypes("/**\n" +
" * @constructor\n" +
" * @dict\n" +
" */" +
"function Dict1() { this['prop'] = 123; }" +
"/** @constructor */" +
"function NonDict() { this.prop = 321; }" +
"/** @param{(NonDict|Dict1)} x */" +
"function takesDict(x) { return x.prop; }",
"Cannot do '.' access on a dict");
}
public void testGetpropDict4() throws Exception {
testTypes("/**\n" +
" * @constructor\n" +
" * @dict\n" +
" */" +
"function Dict1() { this['prop'] = 123; }" +
"/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */" +
"function Struct1() { this.prop = 123; }" +
"/** @param{(Struct1|Dict1)} x */" +
"function takesNothing(x) { return x.prop; }",
"Cannot do '.' access on a dict");
}
public void testGetpropDict5() throws Exception {
testTypes("/**\n" +
" * @constructor\n" +
" * @dict\n" +
" */" +
"function Dict1(){ this.prop = 123; }",
"Cannot do '.' access on a dict");
}
public void testGetpropDict6() throws Exception {
testTypes("/**\n" +
" * @constructor\n" +
" * @dict\n" +
" */\n" +
"function Foo() {}\n" +
"function Bar() {}\n" +
"Bar.prototype = new Foo();\n" +
"Bar.prototype.someprop = 123;\n",
"Cannot do '.' access on a dict");
}
public void testGetpropDict7() throws Exception {
testTypes("(/** @dict */ {x: 123}).x = 321;",
"Cannot do '.' access on a dict");
}
public void testGetelemStruct1() throws Exception {
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */" +
"function Struct1(){ this.prop = 123; }" +
"/** @param{Struct1} x */" +
"function takesStruct(x) {" +
" var z = x;" +
" return z['prop'];" +
"}",
"Cannot do '[]' access on a struct");
}
public void testGetelemStruct2() throws Exception {
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */" +
"function Struct1(){ this.prop = 123; }" +
"/**\n" +
" * @constructor\n" +
" * @extends {Struct1}" +
" */" +
"function Struct1kid(){ this.prop = 123; }" +
"/** @param{Struct1kid} x */" +
"function takesStruct2(x) { return x['prop']; }",
"Cannot do '[]' access on a struct");
}
public void testGetelemStruct3() throws Exception {
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */" +
"function Struct1(){ this.prop = 123; }" +
"/**\n" +
" * @constructor\n" +
" * @extends {Struct1}\n" +
" */" +
"function Struct1kid(){ this.prop = 123; }" +
"var x = (new Struct1kid())['prop'];",
"Cannot do '[]' access on a struct");
}
public void testGetelemStruct4() throws Exception {
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */" +
"function Struct1() { this.prop = 123; }" +
"/** @constructor */" +
"function NonStruct() { this.prop = 321; }" +
"/** @param{(NonStruct|Struct1)} x */" +
"function takesStruct(x) { return x['prop']; }",
"Cannot do '[]' access on a struct");
}
public void testGetelemStruct5() throws Exception {
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */" +
"function Struct1() { this.prop = 123; }" +
"/**\n" +
" * @constructor\n" +
" * @dict\n" +
" */" +
"function Dict1() { this['prop'] = 123; }" +
"/** @param{(Struct1|Dict1)} x */" +
"function takesNothing(x) { return x['prop']; }",
"Cannot do '[]' access on a struct");
}
public void testGetelemStruct6() throws Exception {
// By casting Bar to Foo, the illegal bracket access is not detected
testTypes("/** @interface */ function Foo(){}\n" +
"/**\n" +
" * @constructor\n" +
" * @struct\n" +
" * @implements {Foo}\n" +
" */" +
"function Bar(){ this.x = 123; }\n" +
"var z = /** @type {Foo} */(new Bar)['x'];");
}
public void testGetelemStruct7() throws Exception {
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */\n" +
"function Foo() {}\n" +
"function Bar() {}\n" +
"Bar.prototype = new Foo();\n" +
"Bar.prototype['someprop'] = 123;\n",
"Cannot do '[]' access on a struct");
}
public void testInOnStruct() throws Exception {
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */" +
"function Foo() {}\n" +
"if ('prop' in (new Foo())) {}",
"Cannot use the IN operator with structs");
}
public void testForinOnStruct() throws Exception {
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */" +
"function Foo() {}\n" +
"for (var prop in (new Foo())) {}",
"Cannot use the IN operator with structs");
}
public void testArrayAccess1() throws Exception {
testTypes("var a = []; var b = a['hi'];");
}
public void testArrayAccess2() throws Exception {
testTypes("var a = []; var b = a[[1,2]];",
"array access\n" +
"found : Array\n" +
"required: number");
}
public void testArrayAccess3() throws Exception {
testTypes("var bar = [];" +
"/** @return {void} */function baz(){};" +
"var foo = bar[baz()];",
"array access\n" +
"found : undefined\n" +
"required: number");
}
public void testArrayAccess4() throws Exception {
testTypes("/**@return {!Array}*/function foo(){};var bar = foo()[foo()];",
"array access\n" +
"found : Array\n" +
"required: number");
}
public void testArrayAccess6() throws Exception {
testTypes("var bar = null[1];",
"only arrays or objects can be accessed\n" +
"found : null\n" +
"required: Object");
}
public void testArrayAccess7() throws Exception {
testTypes("var bar = void 0; bar[0];",
"only arrays or objects can be accessed\n" +
"found : undefined\n" +
"required: Object");
}
public void testArrayAccess8() throws Exception {
// Verifies that we don't emit two warnings, because
// the var has been dereferenced after the first one.
testTypes("var bar = void 0; bar[0]; bar[1];",
"only arrays or objects can be accessed\n" +
"found : undefined\n" +
"required: Object");
}
public void testArrayAccess9() throws Exception {
testTypes("/** @return {?Array} */ function f() { return []; }" +
"f()[{}]",
"array access\n" +
"found : {}\n" +
"required: number");
}
public void testPropAccess() throws Exception {
testTypes("/** @param {*} x */var f = function(x) {\n" +
"var o = String(x);\n" +
"if (typeof o['a'] != 'undefined') { return o['a']; }\n" +
"return null;\n" +
"};");
}
public void testPropAccess2() throws Exception {
testTypes("var bar = void 0; bar.baz;",
"No properties on this expression\n" +
"found : undefined\n" +
"required: Object");
}
public void testPropAccess3() throws Exception {
// Verifies that we don't emit two warnings, because
// the var has been dereferenced after the first one.
testTypes("var bar = void 0; bar.baz; bar.bax;",
"No properties on this expression\n" +
"found : undefined\n" +
"required: Object");
}
public void testPropAccess4() throws Exception {
testTypes("/** @param {*} x */ function f(x) { return x['hi']; }");
}
public void testSwitchCase1() throws Exception {
testTypes("/**@type number*/var a;" +
"/**@type string*/var b;" +
"switch(a){case b:;}",
"case expression doesn't match switch\n" +
"found : string\n" +
"required: number");
}
public void testSwitchCase2() throws Exception {
testTypes("var a = null; switch (typeof a) { case 'foo': }");
}
public void testVar1() throws Exception {
TypeCheckResult p =
parseAndTypeCheckWithScope("/** @type {(string,null)} */var a = null");
assertTypeEquals(createUnionType(STRING_TYPE, NULL_TYPE),
p.scope.getVar("a").getType());
}
public void testVar2() throws Exception {
testTypes("/** @type {Function} */ var a = function(){}");
}
public void testVar3() throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope("var a = 3;");
assertTypeEquals(NUMBER_TYPE, p.scope.getVar("a").getType());
}
public void testVar4() throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope(
"var a = 3; a = 'string';");
assertTypeEquals(createUnionType(STRING_TYPE, NUMBER_TYPE),
p.scope.getVar("a").getType());
}
public void testVar5() throws Exception {
testTypes("var goog = {};" +
"/** @type string */goog.foo = 'hello';" +
"/** @type number */var a = goog.foo;",
"initializing variable\n" +
"found : string\n" +
"required: number");
}
public void testVar6() throws Exception {
testTypes(
"function f() {" +
" return function() {" +
" /** @type {!Date} */" +
" var a = 7;" +
" };" +
"}",
"initializing variable\n" +
"found : number\n" +
"required: Date");
}
public void testVar7() throws Exception {
testTypes("/** @type number */var a, b;",
"declaration of multiple variables with shared type information");
}
public void testVar8() throws Exception {
testTypes("var a, b;");
}
public void testVar9() throws Exception {
testTypes("/** @enum */var a;",
"enum initializer must be an object literal or an enum");
}
public void testVar10() throws Exception {
testTypes("/** @type !Number */var foo = 'abc';",
"initializing variable\n" +
"found : string\n" +
"required: Number");
}
public void testVar11() throws Exception {
testTypes("var /** @type !Date */foo = 'abc';",
"initializing variable\n" +
"found : string\n" +
"required: Date");
}
public void testVar12() throws Exception {
testTypes("var /** @type !Date */foo = 'abc', " +
"/** @type !RegExp */bar = 5;",
new String[] {
"initializing variable\n" +
"found : string\n" +
"required: Date",
"initializing variable\n" +
"found : number\n" +
"required: RegExp"});
}
public void testVar13() throws Exception {
// this caused an NPE
testTypes("var /** @type number */a,a;");
}
public void testVar14() throws Exception {
testTypes("/** @return {number} */ function f() { var x; return x; }",
"inconsistent return type\n" +
"found : undefined\n" +
"required: number");
}
public void testVar15() throws Exception {
testTypes("/** @return {number} */" +
"function f() { var x = x || {}; return x; }",
"inconsistent return type\n" +
"found : {}\n" +
"required: number");
}
public void testAssign1() throws Exception {
testTypes("var goog = {};" +
"/** @type number */goog.foo = 'hello';",
"assignment to property foo of goog\n" +
"found : string\n" +
"required: number");
}
public void testAssign2() throws Exception {
testTypes("var goog = {};" +
"/** @type number */goog.foo = 3;" +
"goog.foo = 'hello';",
"assignment to property foo of goog\n" +
"found : string\n" +
"required: number");
}
public void testAssign3() throws Exception {
testTypes("var goog = {};" +
"/** @type number */goog.foo = 3;" +
"goog.foo = 4;");
}
public void testAssign4() throws Exception {
testTypes("var goog = {};" +
"goog.foo = 3;" +
"goog.foo = 'hello';");
}
public void testAssignInference() throws Exception {
testTypes(
"/**" +
" * @param {Array} x" +
" * @return {number}" +
" */" +
"function f(x) {" +
" var y = null;" +
" y = x[0];" +
" if (y == null) { return 4; } else { return 6; }" +
"}");
}
public void testOr1() throws Exception {
testTypes("/** @type number */var a;" +
"/** @type number */var b;" +
"a + b || undefined;");
}
public void testOr2() throws Exception {
testTypes("/** @type number */var a;" +
"/** @type number */var b;" +
"/** @type number */var c = a + b || undefined;",
"initializing variable\n" +
"found : (number|undefined)\n" +
"required: number");
}
public void testOr3() throws Exception {
testTypes("/** @type {(number, undefined)} */var a;" +
"/** @type number */var c = a || 3;");
}
/**
* Test that type inference continues with the right side,
* when no short-circuiting is possible.
* See bugid 1205387 for more details.
*/
public void testOr4() throws Exception {
testTypes("/**@type {number} */var x;x=null || \"a\";",
"assignment\n" +
"found : string\n" +
"required: number");
}
/**
* @see #testOr4()
*/
public void testOr5() throws Exception {
testTypes("/**@type {number} */var x;x=undefined || \"a\";",
"assignment\n" +
"found : string\n" +
"required: number");
}
public void testAnd1() throws Exception {
testTypes("/** @type number */var a;" +
"/** @type number */var b;" +
"a + b && undefined;");
}
public void testAnd2() throws Exception {
testTypes("/** @type number */var a;" +
"/** @type number */var b;" +
"/** @type number */var c = a + b && undefined;",
"initializing variable\n" +
"found : (number|undefined)\n" +
"required: number");
}
public void testAnd3() throws Exception {
testTypes("/** @type {(!Array, undefined)} */var a;" +
"/** @type number */var c = a && undefined;",
"initializing variable\n" +
"found : undefined\n" +
"required: number");
}
public void testAnd4() throws Exception {
testTypes("/** @param {number} x */function f(x){};\n" +
"/** @type null */var x; /** @type {number?} */var y;\n" +
"if (x && y) { f(y) }");
}
public void testAnd5() throws Exception {
testTypes("/** @param {number} x\n@param {string} y*/function f(x,y){};\n" +
"/** @type {number?} */var x; /** @type {string?} */var y;\n" +
"if (x && y) { f(x, y) }");
}
public void testAnd6() throws Exception {
testTypes("/** @param {number} x */function f(x){};\n" +
"/** @type {number|undefined} */var x;\n" +
"if (x && f(x)) { f(x) }");
}
public void testAnd7() throws Exception {
// TODO(user): a deterministic warning should be generated for this
// case since x && x is always false. The implementation of this requires
// a more precise handling of a null value within a variable's type.
// Currently, a null value defaults to ? which passes every check.
testTypes("/** @type null */var x; if (x && x) {}");
}
public void testHook() throws Exception {
testTypes("/**@return {void}*/function foo(){ var x=foo()?a:b; }");
}
public void testHookRestrictsType1() throws Exception {
testTypes("/** @return {(string,null)} */" +
"function f() { return null;}" +
"/** @type {(string,null)} */ var a = f();" +
"/** @type string */" +
"var b = a ? a : 'default';");
}
public void testHookRestrictsType2() throws Exception {
testTypes("/** @type {String} */" +
"var a = null;" +
"/** @type null */" +
"var b = a ? null : a;");
}
public void testHookRestrictsType3() throws Exception {
testTypes("/** @type {String} */" +
"var a;" +
"/** @type null */" +
"var b = (!a) ? a : null;");
}
public void testHookRestrictsType4() throws Exception {
testTypes("/** @type {(boolean,undefined)} */" +
"var a;" +
"/** @type boolean */" +
"var b = a != null ? a : true;");
}
public void testHookRestrictsType5() throws Exception {
testTypes("/** @type {(boolean,undefined)} */" +
"var a;" +
"/** @type {(undefined)} */" +
"var b = a == null ? a : undefined;");
}
public void testHookRestrictsType6() throws Exception {
testTypes("/** @type {(number,null,undefined)} */" +
"var a;" +
"/** @type {number} */" +
"var b = a == null ? 5 : a;");
}
public void testHookRestrictsType7() throws Exception {
testTypes("/** @type {(number,null,undefined)} */" +
"var a;" +
"/** @type {number} */" +
"var b = a == undefined ? 5 : a;");
}
public void testWhileRestrictsType1() throws Exception {
testTypes("/** @param {null} x */ function g(x) {}" +
"/** @param {number?} x */\n" +
"function f(x) {\n" +
"while (x) {\n" +
"if (g(x)) { x = 1; }\n" +
"x = x-1;\n}\n}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : number\n" +
"required: null");
}
public void testWhileRestrictsType2() throws Exception {
testTypes("/** @param {number?} x\n@return {number}*/\n" +
"function f(x) {\n/** @type {number} */var y = 0;" +
"while (x) {\n" +
"y = x;\n" +
"x = x-1;\n}\n" +
"return y;}");
}
public void testHigherOrderFunctions1() throws Exception {
testTypes(
"/** @type {function(number)} */var f;" +
"f(true);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testHigherOrderFunctions2() throws Exception {
testTypes(
"/** @type {function():!Date} */var f;" +
"/** @type boolean */var a = f();",
"initializing variable\n" +
"found : Date\n" +
"required: boolean");
}
public void testHigherOrderFunctions3() throws Exception {
testTypes(
"/** @type {function(this:Error):Date} */var f; new f",
"cannot instantiate non-constructor");
}
public void testHigherOrderFunctions4() throws Exception {
testTypes(
"/** @type {function(this:Error,...[number]):Date} */var f; new f",
"cannot instantiate non-constructor");
}
public void testHigherOrderFunctions5() throws Exception {
testTypes(
"/** @param {number} x */ function g(x) {}" +
"/** @type {function(new:Error,...[number]):Date} */ var f;" +
"g(new f());",
"actual parameter 1 of g does not match formal parameter\n" +
"found : Error\n" +
"required: number");
}
public void testConstructorAlias1() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {};" +
"/** @type {number} */ Foo.prototype.bar = 3;" +
"/** @constructor */ var FooAlias = Foo;" +
"/** @return {string} */ function foo() { " +
" return (new FooAlias()).bar; }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testConstructorAlias2() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {};" +
"/** @constructor */ var FooAlias = Foo;" +
"/** @type {number} */ FooAlias.prototype.bar = 3;" +
"/** @return {string} */ function foo() { " +
" return (new Foo()).bar; }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testConstructorAlias3() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {};" +
"/** @type {number} */ Foo.prototype.bar = 3;" +
"/** @constructor */ var FooAlias = Foo;" +
"/** @return {string} */ function foo() { " +
" return (new FooAlias()).bar; }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testConstructorAlias4() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {};" +
"var FooAlias = Foo;" +
"/** @type {number} */ FooAlias.prototype.bar = 3;" +
"/** @return {string} */ function foo() { " +
" return (new Foo()).bar; }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testConstructorAlias5() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {};" +
"/** @constructor */ var FooAlias = Foo;" +
"/** @return {FooAlias} */ function foo() { " +
" return new Foo(); }");
}
public void testConstructorAlias6() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {};" +
"/** @constructor */ var FooAlias = Foo;" +
"/** @return {Foo} */ function foo() { " +
" return new FooAlias(); }");
}
public void testConstructorAlias7() throws Exception {
testTypes(
"var goog = {};" +
"/** @constructor */ goog.Foo = function() {};" +
"/** @constructor */ goog.FooAlias = goog.Foo;" +
"/** @return {number} */ function foo() { " +
" return new goog.FooAlias(); }",
"inconsistent return type\n" +
"found : goog.Foo\n" +
"required: number");
}
public void testConstructorAlias8() throws Exception {
testTypes(
"var goog = {};" +
"/**\n * @param {number} x \n * @constructor */ " +
"goog.Foo = function(x) {};" +
"/**\n * @param {number} x \n * @constructor */ " +
"goog.FooAlias = goog.Foo;" +
"/** @return {number} */ function foo() { " +
" return new goog.FooAlias(1); }",
"inconsistent return type\n" +
"found : goog.Foo\n" +
"required: number");
}
public void testConstructorAlias9() throws Exception {
testTypes(
"var goog = {};" +
"/**\n * @param {number} x \n * @constructor */ " +
"goog.Foo = function(x) {};" +
"/** @constructor */ goog.FooAlias = goog.Foo;" +
"/** @return {number} */ function foo() { " +
" return new goog.FooAlias(1); }",
"inconsistent return type\n" +
"found : goog.Foo\n" +
"required: number");
}
public void testConstructorAlias10() throws Exception {
testTypes(
"/**\n * @param {number} x \n * @constructor */ " +
"var Foo = function(x) {};" +
"/** @constructor */ var FooAlias = Foo;" +
"/** @return {number} */ function foo() { " +
" return new FooAlias(1); }",
"inconsistent return type\n" +
"found : Foo\n" +
"required: number");
}
public void testClosure1() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {string|undefined} */var a;" +
"/** @type string */" +
"var b = goog.isDef(a) ? a : 'default';",
null);
}
public void testClosure2() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {string?} */var a;" +
"/** @type string */" +
"var b = goog.isNull(a) ? 'default' : a;",
null);
}
public void testClosure3() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {string|null|undefined} */var a;" +
"/** @type string */" +
"var b = goog.isDefAndNotNull(a) ? a : 'default';",
null);
}
public void testClosure4() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {string|undefined} */var a;" +
"/** @type string */" +
"var b = !goog.isDef(a) ? 'default' : a;",
null);
}
public void testClosure5() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {string?} */var a;" +
"/** @type string */" +
"var b = !goog.isNull(a) ? a : 'default';",
null);
}
public void testClosure6() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {string|null|undefined} */var a;" +
"/** @type string */" +
"var b = !goog.isDefAndNotNull(a) ? 'default' : a;",
null);
}
public void testClosure7() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {string|null|undefined} */ var a = foo();" +
"/** @type {number} */" +
"var b = goog.asserts.assert(a);",
"initializing variable\n" +
"found : string\n" +
"required: number");
}
public void testReturn1() throws Exception {
testTypes("/**@return {void}*/function foo(){ return 3; }",
"inconsistent return type\n" +
"found : number\n" +
"required: undefined");
}
public void testReturn2() throws Exception {
testTypes("/**@return {!Number}*/function foo(){ return; }",
"inconsistent return type\n" +
"found : undefined\n" +
"required: Number");
}
public void testReturn3() throws Exception {
testTypes("/**@return {!Number}*/function foo(){ return 'abc'; }",
"inconsistent return type\n" +
"found : string\n" +
"required: Number");
}
public void testReturn4() throws Exception {
testTypes("/**@return {!Number}\n*/\n function a(){return new Array();}",
"inconsistent return type\n" +
"found : Array\n" +
"required: Number");
}
public void testReturn5() throws Exception {
testTypes("/** @param {number} n\n" +
"@constructor */function n(n){return};");
}
public void testReturn6() throws Exception {
testTypes(
"/** @param {number} opt_a\n@return {string} */" +
"function a(opt_a) { return opt_a }",
"inconsistent return type\n" +
"found : (number|undefined)\n" +
"required: string");
}
public void testReturn7() throws Exception {
testTypes("/** @constructor */var A = function() {};\n" +
"/** @constructor */var B = function() {};\n" +
"/** @return {!B} */A.f = function() { return 1; };",
"inconsistent return type\n" +
"found : number\n" +
"required: B");
}
public void testReturn8() throws Exception {
testTypes("/** @constructor */var A = function() {};\n" +
"/** @constructor */var B = function() {};\n" +
"/** @return {!B} */A.prototype.f = function() { return 1; };",
"inconsistent return type\n" +
"found : number\n" +
"required: B");
}
public void testInferredReturn1() throws Exception {
testTypes(
"function f() {} /** @param {number} x */ function g(x) {}" +
"g(f());",
"actual parameter 1 of g does not match formal parameter\n" +
"found : undefined\n" +
"required: number");
}
public void testInferredReturn2() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() {}; " +
"/** @param {number} x */ function g(x) {}" +
"g((new Foo()).bar());",
"actual parameter 1 of g does not match formal parameter\n" +
"found : undefined\n" +
"required: number");
}
public void testInferredReturn3() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() {}; " +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @return {number} \n * @override */ " +
"SubFoo.prototype.bar = function() { return 3; }; ",
"mismatch of the bar property type and the type of the property " +
"it overrides from superclass Foo\n" +
"original: function (this:Foo): undefined\n" +
"override: function (this:SubFoo): number");
}
public void testInferredReturn4() throws Exception {
// By design, this throws a warning. if you want global x to be
// defined to some other type of function, then you need to declare it
// as a greater type.
testTypes(
"var x = function() {};" +
"x = /** @type {function(): number} */ (function() { return 3; });",
"assignment\n" +
"found : function (): number\n" +
"required: function (): undefined");
}
public void testInferredReturn5() throws Exception {
// If x is local, then the function type is not declared.
testTypes(
"/** @return {string} */" +
"function f() {" +
" var x = function() {};" +
" x = /** @type {function(): number} */ (function() { return 3; });" +
" return x();" +
"}",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testInferredReturn6() throws Exception {
testTypes(
"/** @return {string} */" +
"function f() {" +
" var x = function() {};" +
" if (f()) " +
" x = /** @type {function(): number} */ " +
" (function() { return 3; });" +
" return x();" +
"}",
"inconsistent return type\n" +
"found : (number|undefined)\n" +
"required: string");
}
public void testInferredReturn7() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @param {number} x */ Foo.prototype.bar = function(x) {};" +
"Foo.prototype.bar = function(x) { return 3; };",
"inconsistent return type\n" +
"found : number\n" +
"required: undefined");
}
public void testInferredReturn8() throws Exception {
reportMissingOverrides = CheckLevel.OFF;
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @param {number} x */ Foo.prototype.bar = function(x) {};" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @param {number} x */ SubFoo.prototype.bar = " +
" function(x) { return 3; }",
"inconsistent return type\n" +
"found : number\n" +
"required: undefined");
}
public void testInferredParam1() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @param {number} x */ Foo.prototype.bar = function(x) {};" +
"/** @param {string} x */ function f(x) {}" +
"Foo.prototype.bar = function(y) { f(y); };",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testInferredParam2() throws Exception {
reportMissingOverrides = CheckLevel.OFF;
testTypes(
"/** @param {string} x */ function f(x) {}" +
"/** @constructor */ function Foo() {}" +
"/** @param {number} x */ Foo.prototype.bar = function(x) {};" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @return {void} */ SubFoo.prototype.bar = " +
" function(x) { f(x); }",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testInferredParam3() throws Exception {
reportMissingOverrides = CheckLevel.OFF;
testTypes(
"/** @param {string} x */ function f(x) {}" +
"/** @constructor */ function Foo() {}" +
"/** @param {number=} x */ Foo.prototype.bar = function(x) {};" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @return {void} */ SubFoo.prototype.bar = " +
" function(x) { f(x); }; (new SubFoo()).bar();",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (number|undefined)\n" +
"required: string");
}
public void testInferredParam4() throws Exception {
reportMissingOverrides = CheckLevel.OFF;
testTypes(
"/** @param {string} x */ function f(x) {}" +
"/** @constructor */ function Foo() {}" +
"/** @param {...number} x */ Foo.prototype.bar = function(x) {};" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @return {void} */ SubFoo.prototype.bar = " +
" function(x) { f(x); }; (new SubFoo()).bar();",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (number|undefined)\n" +
"required: string");
}
public void testInferredParam5() throws Exception {
reportMissingOverrides = CheckLevel.OFF;
testTypes(
"/** @param {string} x */ function f(x) {}" +
"/** @constructor */ function Foo() {}" +
"/** @param {...number} x */ Foo.prototype.bar = function(x) {};" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @param {number=} x \n * @param {...number} y */ " +
"SubFoo.prototype.bar = " +
" function(x, y) { f(x); }; (new SubFoo()).bar();",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (number|undefined)\n" +
"required: string");
}
public void testInferredParam6() throws Exception {
reportMissingOverrides = CheckLevel.OFF;
testTypes(
"/** @param {string} x */ function f(x) {}" +
"/** @constructor */ function Foo() {}" +
"/** @param {number=} x */ Foo.prototype.bar = function(x) {};" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @param {number=} x \n * @param {number=} y */ " +
"SubFoo.prototype.bar = " +
" function(x, y) { f(y); };",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (number|undefined)\n" +
"required: string");
}
public void testInferredParam7() throws Exception {
testTypes(
"/** @param {string} x */ function f(x) {}" +
"var bar = /** @type {function(number=,number=)} */ (" +
" function(x, y) { f(y); });",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (number|undefined)\n" +
"required: string");
}
public void testOverriddenParams1() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @param {...?} var_args */" +
"Foo.prototype.bar = function(var_args) {};" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/**\n" +
" * @param {number} x\n" +
" * @override\n" +
" */" +
"SubFoo.prototype.bar = function(x) {};");
}
public void testOverriddenParams2() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @type {function(...[?])} */" +
"Foo.prototype.bar = function(var_args) {};" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/**\n" +
" * @type {function(number)}\n" +
" * @override\n" +
" */" +
"SubFoo.prototype.bar = function(x) {};");
}
public void testOverriddenParams3() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @param {...number} var_args */" +
"Foo.prototype.bar = function(var_args) { };" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/**\n" +
" * @param {number} x\n" +
" * @override\n" +
" */" +
"SubFoo.prototype.bar = function(x) {};",
"mismatch of the bar property type and the type of the " +
"property it overrides from superclass Foo\n" +
"original: function (this:Foo, ...[number]): undefined\n" +
"override: function (this:SubFoo, number): undefined");
}
public void testOverriddenParams4() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @type {function(...[number])} */" +
"Foo.prototype.bar = function(var_args) {};" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/**\n" +
" * @type {function(number)}\n" +
" * @override\n" +
" */" +
"SubFoo.prototype.bar = function(x) {};",
"mismatch of the bar property type and the type of the " +
"property it overrides from superclass Foo\n" +
"original: function (...[number]): ?\n" +
"override: function (number): ?");
}
public void testOverriddenParams5() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @param {number} x */" +
"Foo.prototype.bar = function(x) { };" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/**\n" +
" * @override\n" +
" */" +
"SubFoo.prototype.bar = function() {};" +
"(new SubFoo()).bar();");
}
public void testOverriddenParams6() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @param {number} x */" +
"Foo.prototype.bar = function(x) { };" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/**\n" +
" * @override\n" +
" */" +
"SubFoo.prototype.bar = function() {};" +
"(new SubFoo()).bar(true);",
"actual parameter 1 of SubFoo.prototype.bar " +
"does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testOverriddenReturn1() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @return {Object} */ Foo.prototype.bar = " +
" function() { return {}; };" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @return {SubFoo}\n * @override */ SubFoo.prototype.bar = " +
" function() { return new Foo(); }",
"inconsistent return type\n" +
"found : Foo\n" +
"required: (SubFoo|null)");
}
public void testOverriddenReturn2() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @return {SubFoo} */ Foo.prototype.bar = " +
" function() { return new SubFoo(); };" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @return {Foo} x\n * @override */ SubFoo.prototype.bar = " +
" function() { return new SubFoo(); }",
"mismatch of the bar property type and the type of the " +
"property it overrides from superclass Foo\n" +
"original: function (this:Foo): (SubFoo|null)\n" +
"override: function (this:SubFoo): (Foo|null)");
}
public void testThis1() throws Exception {
testTypes("var goog = {};" +
"/** @constructor */goog.A = function(){};" +
"/** @return {number} */" +
"goog.A.prototype.n = function() { return this };",
"inconsistent return type\n" +
"found : goog.A\n" +
"required: number");
}
public void testOverriddenProperty1() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @type {Object} */" +
"Foo.prototype.bar = {};" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/**\n" +
" * @type {Array}\n" +
" * @override\n" +
" */" +
"SubFoo.prototype.bar = [];");
}
public void testOverriddenProperty2() throws Exception {
testTypes(
"/** @constructor */ function Foo() {" +
" /** @type {Object} */" +
" this.bar = {};" +
"}" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/**\n" +
" * @type {Array}\n" +
" * @override\n" +
" */" +
"SubFoo.prototype.bar = [];");
}
public void testOverriddenProperty3() throws Exception {
testTypes(
"/** @constructor */ function Foo() {" +
"}" +
"/** @type {string} */ Foo.prototype.data;" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/** @type {string|Object} \n @override */ " +
"SubFoo.prototype.data = null;",
"mismatch of the data property type and the type " +
"of the property it overrides from superclass Foo\n" +
"original: string\n" +
"override: (Object|null|string)");
}
public void testOverriddenProperty4() throws Exception {
// These properties aren't declared, so there should be no warning.
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = null;" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"SubFoo.prototype.bar = 3;");
}
public void testOverriddenProperty5() throws Exception {
// An override should be OK if the superclass property wasn't declared.
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = null;" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/** @override */ SubFoo.prototype.bar = 3;");
}
public void testOverriddenProperty6() throws Exception {
// The override keyword shouldn't be neccessary if the subclass property
// is inferred.
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @type {?number} */ Foo.prototype.bar = null;" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"SubFoo.prototype.bar = 3;");
}
public void testThis2() throws Exception {
testTypes("var goog = {};" +
"/** @constructor */goog.A = function(){" +
" this.foo = null;" +
"};" +
"/** @return {number} */" +
"goog.A.prototype.n = function() { return this.foo };",
"inconsistent return type\n" +
"found : null\n" +
"required: number");
}
public void testThis3() throws Exception {
testTypes("var goog = {};" +
"/** @constructor */goog.A = function(){" +
" this.foo = null;" +
" this.foo = 5;" +
"};");
}
public void testThis4() throws Exception {
testTypes("var goog = {};" +
"/** @constructor */goog.A = function(){" +
" /** @type {string?} */this.foo = null;" +
"};" +
"/** @return {number} */goog.A.prototype.n = function() {" +
" return this.foo };",
"inconsistent return type\n" +
"found : (null|string)\n" +
"required: number");
}
public void testThis5() throws Exception {
testTypes("/** @this Date\n@return {number}*/function h() { return this }",
"inconsistent return type\n" +
"found : Date\n" +
"required: number");
}
public void testThis6() throws Exception {
testTypes("var goog = {};" +
"/** @constructor\n@return {!Date} */" +
"goog.A = function(){ return this };",
"inconsistent return type\n" +
"found : goog.A\n" +
"required: Date");
}
public void testThis7() throws Exception {
testTypes("/** @constructor */function A(){};" +
"/** @return {number} */A.prototype.n = function() { return this };",
"inconsistent return type\n" +
"found : A\n" +
"required: number");
}
public void testThis8() throws Exception {
testTypes("/** @constructor */function A(){" +
" /** @type {string?} */this.foo = null;" +
"};" +
"/** @return {number} */A.prototype.n = function() {" +
" return this.foo };",
"inconsistent return type\n" +
"found : (null|string)\n" +
"required: number");
}
public void testThis9() throws Exception {
// In A.bar, the type of {@code this} is unknown.
testTypes("/** @constructor */function A(){};" +
"A.prototype.foo = 3;" +
"/** @return {string} */ A.bar = function() { return this.foo; };");
}
public void testThis10() throws Exception {
// In A.bar, the type of {@code this} is inferred from the @this tag.
testTypes("/** @constructor */function A(){};" +
"A.prototype.foo = 3;" +
"/** @this {A}\n@return {string} */" +
"A.bar = function() { return this.foo; };",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testThis11() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"/** @constructor */ function Ctor() {" +
" /** @this {Date} */" +
" this.method = function() {" +
" f(this);" +
" };" +
"}",
"actual parameter 1 of f does not match formal parameter\n" +
"found : Date\n" +
"required: number");
}
public void testThis12() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"/** @constructor */ function Ctor() {}" +
"Ctor.prototype['method'] = function() {" +
" f(this);" +
"}",
"actual parameter 1 of f does not match formal parameter\n" +
"found : Ctor\n" +
"required: number");
}
public void testThis13() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"/** @constructor */ function Ctor() {}" +
"Ctor.prototype = {" +
" method: function() {" +
" f(this);" +
" }" +
"};",
"actual parameter 1 of f does not match formal parameter\n" +
"found : Ctor\n" +
"required: number");
}
public void testThis14() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"f(this.Object);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : function (new:Object, *=): ?\n" +
"required: number");
}
public void testThisTypeOfFunction1() throws Exception {
testTypes(
"/** @type {function(this:Object)} */ function f() {}" +
"f();");
}
public void testThisTypeOfFunction2() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** @type {function(this:F)} */ function f() {}" +
"f();",
"\"function (this:F): ?\" must be called with a \"this\" type");
}
public void testThisTypeOfFunction3() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"F.prototype.bar = function() {};" +
"var f = (new F()).bar; f();",
"\"function (this:F): undefined\" must be called with a \"this\" type");
}
public void testThisTypeOfFunction4() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"F.prototype.moveTo = function(x, y) {};" +
"F.prototype.lineTo = function(x, y) {};" +
"function demo() {" +
" var path = new F();" +
" var points = [[1,1], [2,2]];" +
" for (var i = 0; i < points.length; i++) {" +
" (i == 0 ? path.moveTo : path.lineTo)(" +
" points[i][0], points[i][1]);" +
" }" +
"}",
"\"function (this:F, ?, ?): undefined\" " +
"must be called with a \"this\" type");
}
public void testGlobalThis1() throws Exception {
testTypes("/** @constructor */ function Window() {}" +
"/** @param {string} msg */ " +
"Window.prototype.alert = function(msg) {};" +
"this.alert(3);",
"actual parameter 1 of Window.prototype.alert " +
"does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testGlobalThis2() throws Exception {
// this.alert = 3 doesn't count as a declaration, so this isn't a warning.
testTypes("/** @constructor */ function Bindow() {}" +
"/** @param {string} msg */ " +
"Bindow.prototype.alert = function(msg) {};" +
"this.alert = 3;" +
"(new Bindow()).alert(this.alert)");
}
public void testGlobalThis2b() throws Exception {
testTypes("/** @constructor */ function Bindow() {}" +
"/** @param {string} msg */ " +
"Bindow.prototype.alert = function(msg) {};" +
"/** @return {number} */ this.alert = function() { return 3; };" +
"(new Bindow()).alert(this.alert())",
"actual parameter 1 of Bindow.prototype.alert " +
"does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testGlobalThis3() throws Exception {
testTypes(
"/** @param {string} msg */ " +
"function alert(msg) {};" +
"this.alert(3);",
"actual parameter 1 of global this.alert " +
"does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testGlobalThis4() throws Exception {
testTypes(
"/** @param {string} msg */ " +
"var alert = function(msg) {};" +
"this.alert(3);",
"actual parameter 1 of global this.alert " +
"does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testGlobalThis5() throws Exception {
testTypes(
"function f() {" +
" /** @param {string} msg */ " +
" var alert = function(msg) {};" +
"}" +
"this.alert(3);",
"Property alert never defined on global this");
}
public void testGlobalThis6() throws Exception {
testTypes(
"/** @param {string} msg */ " +
"var alert = function(msg) {};" +
"var x = 3;" +
"x = 'msg';" +
"this.alert(this.x);");
}
public void testGlobalThis7() throws Exception {
testTypes(
"/** @constructor */ function Window() {}" +
"/** @param {Window} msg */ " +
"var foo = function(msg) {};" +
"foo(this);");
}
public void testGlobalThis8() throws Exception {
testTypes(
"/** @constructor */ function Window() {}" +
"/** @param {number} msg */ " +
"var foo = function(msg) {};" +
"foo(this);",
"actual parameter 1 of foo does not match formal parameter\n" +
"found : global this\n" +
"required: number");
}
public void testGlobalThis9() throws Exception {
testTypes(
// Window is not marked as a constructor, so the
// inheritance doesn't happen.
"function Window() {}" +
"Window.prototype.alert = function() {};" +
"this.alert();",
"Property alert never defined on global this");
}
public void testControlFlowRestrictsType1() throws Exception {
testTypes("/** @return {String?} */ function f() { return null; }" +
"/** @type {String?} */ var a = f();" +
"/** @type String */ var b = new String('foo');" +
"/** @type null */ var c = null;" +
"if (a) {" +
" b = a;" +
"} else {" +
" c = a;" +
"}");
}
public void testControlFlowRestrictsType2() throws Exception {
testTypes("/** @return {(string,null)} */ function f() { return null; }" +
"/** @type {(string,null)} */ var a = f();" +
"/** @type string */ var b = 'foo';" +
"/** @type null */ var c = null;" +
"if (a) {" +
" b = a;" +
"} else {" +
" c = a;" +
"}",
"assignment\n" +
"found : (null|string)\n" +
"required: null");
}
public void testControlFlowRestrictsType3() throws Exception {
testTypes("/** @type {(string,void)} */" +
"var a;" +
"/** @type string */" +
"var b = 'foo';" +
"if (a) {" +
" b = a;" +
"}");
}
public void testControlFlowRestrictsType4() throws Exception {
testTypes("/** @param {string} a */ function f(a){}" +
"/** @type {(string,undefined)} */ var a;" +
"a && f(a);");
}
public void testControlFlowRestrictsType5() throws Exception {
testTypes("/** @param {undefined} a */ function f(a){}" +
"/** @type {(!Array,undefined)} */ var a;" +
"a || f(a);");
}
public void testControlFlowRestrictsType6() throws Exception {
testTypes("/** @param {undefined} x */ function f(x) {}" +
"/** @type {(string,undefined)} */ var a;" +
"a && f(a);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: undefined");
}
public void testControlFlowRestrictsType7() throws Exception {
testTypes("/** @param {undefined} x */ function f(x) {}" +
"/** @type {(string,undefined)} */ var a;" +
"a && f(a);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: undefined");
}
public void testControlFlowRestrictsType8() throws Exception {
testTypes("/** @param {undefined} a */ function f(a){}" +
"/** @type {(!Array,undefined)} */ var a;" +
"if (a || f(a)) {}");
}
public void testControlFlowRestrictsType9() throws Exception {
testTypes("/** @param {number?} x\n * @return {number}*/\n" +
"var f = function(x) {\n" +
"if (!x || x == 1) { return 1; } else { return x; }\n" +
"};");
}
public void testControlFlowRestrictsType10() throws Exception {
// We should correctly infer that y will be (null|{}) because
// the loop wraps around.
testTypes("/** @param {number} x */ function f(x) {}" +
"function g() {" +
" var y = null;" +
" for (var i = 0; i < 10; i++) {" +
" f(y);" +
" if (y != null) {" +
" // y is None the first time it goes through this branch\n" +
" } else {" +
" y = {};" +
" }" +
" }" +
"};",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (null|{})\n" +
"required: number");
}
public void testControlFlowRestrictsType11() throws Exception {
testTypes("/** @param {boolean} x */ function f(x) {}" +
"function g() {" +
" var y = null;" +
" if (y != null) {" +
" for (var i = 0; i < 10; i++) {" +
" f(y);" +
" }" +
" }" +
"};",
"condition always evaluates to false\n" +
"left : null\n" +
"right: null");
}
public void testSwitchCase3() throws Exception {
testTypes("/** @type String */" +
"var a = new String('foo');" +
"switch (a) { case 'A': }");
}
public void testSwitchCase4() throws Exception {
testTypes("/** @type {(string,Null)} */" +
"var a = 'foo';" +
"switch (a) { case 'A':break; case null:break; }");
}
public void testSwitchCase5() throws Exception {
testTypes("/** @type {(String,Null)} */" +
"var a = new String('foo');" +
"switch (a) { case 'A':break; case null:break; }");
}
public void testSwitchCase6() throws Exception {
testTypes("/** @type {(Number,Null)} */" +
"var a = new Number(5);" +
"switch (a) { case 5:break; case null:break; }");
}
public void testSwitchCase7() throws Exception {
// This really tests the inference inside the case.
testTypes(
"/**\n" +
" * @param {number} x\n" +
" * @return {number}\n" +
" */\n" +
"function g(x) { return 5; }" +
"function f() {" +
" var x = {};" +
" x.foo = '3';" +
" switch (3) { case g(x.foo): return 3; }" +
"}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testSwitchCase8() throws Exception {
// This really tests the inference inside the switch clause.
testTypes(
"/**\n" +
" * @param {number} x\n" +
" * @return {number}\n" +
" */\n" +
"function g(x) { return 5; }" +
"function f() {" +
" var x = {};" +
" x.foo = '3';" +
" switch (g(x.foo)) { case 3: return 3; }" +
"}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testNoTypeCheck1() throws Exception {
testTypes("/** @notypecheck */function foo() { new 4 }");
}
public void testNoTypeCheck2() throws Exception {
testTypes("/** @notypecheck */var foo = function() { new 4 }");
}
public void testNoTypeCheck3() throws Exception {
testTypes("/** @notypecheck */var foo = function bar() { new 4 }");
}
public void testNoTypeCheck4() throws Exception {
testTypes("var foo;" +
"/** @notypecheck */foo = function() { new 4 }");
}
public void testNoTypeCheck5() throws Exception {
testTypes("var foo;" +
"foo = /** @notypecheck */function() { new 4 }");
}
public void testNoTypeCheck6() throws Exception {
testTypes("var foo;" +
"/** @notypecheck */foo = function bar() { new 4 }");
}
public void testNoTypeCheck7() throws Exception {
testTypes("var foo;" +
"foo = /** @notypecheck */function bar() { new 4 }");
}
public void testNoTypeCheck8() throws Exception {
testTypes("/** @fileoverview \n * @notypecheck */ var foo;" +
"var bar = 3; /** @param {string} x */ function f(x) {} f(bar);");
}
public void testNoTypeCheck9() throws Exception {
testTypes("/** @notypecheck */ function g() { }" +
" /** @type {string} */ var a = 1",
"initializing variable\n" +
"found : number\n" +
"required: string"
);
}
public void testNoTypeCheck10() throws Exception {
testTypes("/** @notypecheck */ function g() { }" +
" function h() {/** @type {string} */ var a = 1}",
"initializing variable\n" +
"found : number\n" +
"required: string"
);
}
public void testNoTypeCheck11() throws Exception {
testTypes("/** @notypecheck */ function g() { }" +
"/** @notypecheck */ function h() {/** @type {string} */ var a = 1}"
);
}
public void testNoTypeCheck12() throws Exception {
testTypes("/** @notypecheck */ function g() { }" +
"function h() {/** @type {string}\n * @notypecheck\n*/ var a = 1}"
);
}
public void testNoTypeCheck13() throws Exception {
testTypes("/** @notypecheck */ function g() { }" +
"function h() {/** @type {string}\n * @notypecheck\n*/ var a = 1;" +
"/** @type {string}*/ var b = 1}",
"initializing variable\n" +
"found : number\n" +
"required: string"
);
}
public void testNoTypeCheck14() throws Exception {
testTypes("/** @fileoverview \n * @notypecheck */ function g() { }" +
"g(1,2,3)");
}
public void testImplicitCast() throws Exception {
testTypes("/** @constructor */ function Element() {};\n" +
"/** @type {string}\n" +
" * @implicitCast */" +
"Element.prototype.innerHTML;",
"(new Element).innerHTML = new Array();", null, false);
}
public void testImplicitCastSubclassAccess() throws Exception {
testTypes("/** @constructor */ function Element() {};\n" +
"/** @type {string}\n" +
" * @implicitCast */" +
"Element.prototype.innerHTML;" +
"/** @constructor \n @extends Element */" +
"function DIVElement() {};",
"(new DIVElement).innerHTML = new Array();",
null, false);
}
public void testImplicitCastNotInExterns() throws Exception {
testTypes("/** @constructor */ function Element() {};\n" +
"/** @type {string}\n" +
" * @implicitCast */" +
"Element.prototype.innerHTML;" +
"(new Element).innerHTML = new Array();",
new String[] {
"Illegal annotation on innerHTML. @implicitCast may only be " +
"used in externs.",
"assignment to property innerHTML of Element\n" +
"found : Array\n" +
"required: string"});
}
public void testNumberNode() throws Exception {
Node n = typeCheck(Node.newNumber(0));
assertTypeEquals(NUMBER_TYPE, n.getJSType());
}
public void testStringNode() throws Exception {
Node n = typeCheck(Node.newString("hello"));
assertTypeEquals(STRING_TYPE, n.getJSType());
}
public void testBooleanNodeTrue() throws Exception {
Node trueNode = typeCheck(new Node(Token.TRUE));
assertTypeEquals(BOOLEAN_TYPE, trueNode.getJSType());
}
public void testBooleanNodeFalse() throws Exception {
Node falseNode = typeCheck(new Node(Token.FALSE));
assertTypeEquals(BOOLEAN_TYPE, falseNode.getJSType());
}
public void testUndefinedNode() throws Exception {
Node p = new Node(Token.ADD);
Node n = Node.newString(Token.NAME, "undefined");
p.addChildToBack(n);
p.addChildToBack(Node.newNumber(5));
typeCheck(p);
assertTypeEquals(VOID_TYPE, n.getJSType());
}
public void testNumberAutoboxing() throws Exception {
testTypes("/** @type Number */var a = 4;",
"initializing variable\n" +
"found : number\n" +
"required: (Number|null)");
}
public void testNumberUnboxing() throws Exception {
testTypes("/** @type number */var a = new Number(4);",
"initializing variable\n" +
"found : Number\n" +
"required: number");
}
public void testStringAutoboxing() throws Exception {
testTypes("/** @type String */var a = 'hello';",
"initializing variable\n" +
"found : string\n" +
"required: (String|null)");
}
public void testStringUnboxing() throws Exception {
testTypes("/** @type string */var a = new String('hello');",
"initializing variable\n" +
"found : String\n" +
"required: string");
}
public void testBooleanAutoboxing() throws Exception {
testTypes("/** @type Boolean */var a = true;",
"initializing variable\n" +
"found : boolean\n" +
"required: (Boolean|null)");
}
public void testBooleanUnboxing() throws Exception {
testTypes("/** @type boolean */var a = new Boolean(false);",
"initializing variable\n" +
"found : Boolean\n" +
"required: boolean");
}
public void testIIFE1() throws Exception {
testTypes(
"var namespace = {};" +
"/** @type {number} */ namespace.prop = 3;" +
"(function(ns) {" +
" ns.prop = true;" +
"})(namespace);",
"assignment to property prop of ns\n" +
"found : boolean\n" +
"required: number");
}
public void testIIFE2() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"(function(ctor) {" +
" /** @type {boolean} */ ctor.prop = true;" +
"})(Foo);" +
"/** @return {number} */ function f() { return Foo.prop; }",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testIIFE3() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"(function(ctor) {" +
" /** @type {boolean} */ ctor.prop = true;" +
"})(Foo);" +
"/** @param {number} x */ function f(x) {}" +
"f(Foo.prop);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testIIFE4() throws Exception {
testTypes(
"/** @const */ var namespace = {};" +
"(function(ns) {" +
" /**\n" +
" * @constructor\n" +
" * @param {number} x\n" +
" */\n" +
" ns.Ctor = function(x) {};" +
"})(namespace);" +
"new namespace.Ctor(true);",
"actual parameter 1 of namespace.Ctor " +
"does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testIIFE5() throws Exception {
// TODO(nicksantos): This behavior is currently incorrect.
// To handle this case properly, we'll need to change how we handle
// type resolution.
testTypes(
"/** @const */ var namespace = {};" +
"(function(ns) {" +
" /**\n" +
" * @constructor\n" +
" */\n" +
" ns.Ctor = function() {};" +
" /** @type {boolean} */ ns.Ctor.prototype.bar = true;" +
"})(namespace);" +
"/** @param {namespace.Ctor} x\n" +
" * @return {number} */ function f(x) { return x.bar; }",
"Bad type annotation. Unknown type namespace.Ctor");
}
public void testNotIIFE1() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"/** @param {...?} x */ function g(x) {}" +
"g(function(y) { f(y); }, true);");
}
public void testIssue61() throws Exception {
testTypes(
"var ns = {};" +
"(function() {" +
" /** @param {string} b */" +
" ns.a = function(b) {};" +
"})();" +
"function d() {" +
" ns.a(123);" +
"}",
"actual parameter 1 of ns.a does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testIssue61b() throws Exception {
testTypes(
"var ns = {};" +
"(function() {" +
" /** @param {string} b */" +
" ns.a = function(b) {};" +
"})();" +
"ns.a(123);",
"actual parameter 1 of ns.a does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testIssue86() throws Exception {
testTypes(
"/** @interface */ function I() {}" +
"/** @return {number} */ I.prototype.get = function(){};" +
"/** @constructor \n * @implements {I} */ function F() {}" +
"/** @override */ F.prototype.get = function() { return true; };",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testIssue124() throws Exception {
testTypes(
"var t = null;" +
"function test() {" +
" if (t != null) { t = null; }" +
" t = 1;" +
"}");
}
public void testIssue124b() throws Exception {
testTypes(
"var t = null;" +
"function test() {" +
" if (t != null) { t = null; }" +
" t = undefined;" +
"}",
"condition always evaluates to false\n" +
"left : (null|undefined)\n" +
"right: null");
}
public void testIssue259() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"/** @constructor */" +
"var Clock = function() {" +
" /** @constructor */" +
" this.Date = function() {};" +
" f(new this.Date());" +
"};",
"actual parameter 1 of f does not match formal parameter\n" +
"found : this.Date\n" +
"required: number");
}
public void testIssue301() throws Exception {
testTypes(
"Array.indexOf = function() {};" +
"var s = 'hello';" +
"alert(s.toLowerCase.indexOf('1'));",
"Property indexOf never defined on String.prototype.toLowerCase");
}
public void testIssue368() throws Exception {
testTypes(
"/** @constructor */ function Foo(){}" +
"/**\n" +
" * @param {number} one\n" +
" * @param {string} two\n" +
" */\n" +
"Foo.prototype.add = function(one, two) {};" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */\n" +
"function Bar(){}" +
"/** @override */\n" +
"Bar.prototype.add = function(ignored) {};" +
"(new Bar()).add(1, 2);",
"actual parameter 2 of Bar.prototype.add does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testIssue380() throws Exception {
testTypes(
"/** @type { function(string): {innerHTML: string} } */\n" +
"document.getElementById;\n" +
"var list = /** @type {!Array.<string>} */ ['hello', 'you'];\n" +
"list.push('?');\n" +
"document.getElementById('node').innerHTML = list.toString();",
// Parse warning, but still applied.
"Type annotations are not allowed here. " +
"Are you missing parentheses?");
}
public void testIssue483() throws Exception {
testTypes(
"/** @constructor */ function C() {" +
" /** @type {?Array} */ this.a = [];" +
"}" +
"C.prototype.f = function() {" +
" if (this.a.length > 0) {" +
" g(this.a);" +
" }" +
"};" +
"/** @param {number} a */ function g(a) {}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : Array\n" +
"required: number");
}
public void testIssue537a() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype = {method: function() {}};" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */\n" +
"function Bar() {" +
" Foo.call(this);" +
" if (this.baz()) this.method(1);" +
"}" +
"Bar.prototype = {" +
" baz: function() {" +
" return true;" +
" }" +
"};" +
"Bar.prototype.__proto__ = Foo.prototype;",
"Function Foo.prototype.method: called with 1 argument(s). " +
"Function requires at least 0 argument(s) " +
"and no more than 0 argument(s).");
}
public void testIssue537b() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype = {method: function() {}};" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */\n" +
"function Bar() {" +
" Foo.call(this);" +
" if (this.baz(1)) this.method();" +
"}" +
"Bar.prototype = {" +
" baz: function() {" +
" return true;" +
" }" +
"};" +
"Bar.prototype.__proto__ = Foo.prototype;",
"Function Bar.prototype.baz: called with 1 argument(s). " +
"Function requires at least 0 argument(s) " +
"and no more than 0 argument(s).");
}
public void testIssue537c() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */\n" +
"function Bar() {" +
" Foo.call(this);" +
" if (this.baz2()) alert(1);" +
"}" +
"Bar.prototype = {" +
" baz: function() {" +
" return true;" +
" }" +
"};" +
"Bar.prototype.__proto__ = Foo.prototype;",
"Property baz2 never defined on Bar");
}
public void testIssue537d() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype = {" +
" /** @return {Bar} */ x: function() { new Bar(); }," +
" /** @return {Foo} */ y: function() { new Bar(); }" +
"};" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */\n" +
"function Bar() {" +
" this.xy = 3;" +
"}" +
"/** @return {Bar} */ function f() { return new Bar(); }" +
"/** @return {Foo} */ function g() { return new Bar(); }" +
"Bar.prototype = {" +
" /** @return {Bar} */ x: function() { new Bar(); }," +
" /** @return {Foo} */ y: function() { new Bar(); }" +
"};" +
"Bar.prototype.__proto__ = Foo.prototype;");
}
public void testIssue586() throws Exception {
testTypes(
"/** @constructor */" +
"var MyClass = function() {};" +
"/** @param {boolean} success */" +
"MyClass.prototype.fn = function(success) {};" +
"MyClass.prototype.test = function() {" +
" this.fn();" +
" this.fn = function() {};" +
"};",
"Function MyClass.prototype.fn: called with 0 argument(s). " +
"Function requires at least 1 argument(s) " +
"and no more than 1 argument(s).");
}
public void testIssue635() throws Exception {
// TODO(nicksantos): Make this emit a warning, because of the 'this' type.
testTypes(
"/** @constructor */" +
"function F() {}" +
"F.prototype.bar = function() { this.baz(); };" +
"F.prototype.baz = function() {};" +
"/** @constructor */" +
"function G() {}" +
"G.prototype.bar = F.prototype.bar;");
}
public void testIssue635b() throws Exception {
testTypes(
"/** @constructor */" +
"function F() {}" +
"/** @constructor */" +
"function G() {}" +
"/** @type {function(new:G)} */ var x = F;",
"initializing variable\n" +
"found : function (new:F): undefined\n" +
"required: function (new:G): ?");
}
public void testIssue669() throws Exception {
testTypes(
"/** @return {{prop1: (Object|undefined)}} */" +
"function f(a) {" +
" var results;" +
" if (a) {" +
" results = {};" +
" results.prop1 = {a: 3};" +
" } else {" +
" results = {prop2: 3};" +
" }" +
" return results;" +
"}");
}
public void testIssue688() throws Exception {
testTypes(
"/** @const */ var SOME_DEFAULT =\n" +
" /** @type {TwoNumbers} */ ({first: 1, second: 2});\n" +
"/**\n" +
"* Class defining an interface with two numbers.\n" +
"* @interface\n" +
"*/\n" +
"function TwoNumbers() {}\n" +
"/** @type number */\n" +
"TwoNumbers.prototype.first;\n" +
"/** @type number */\n" +
"TwoNumbers.prototype.second;\n" +
"/** @return {number} */ function f() { return SOME_DEFAULT; }",
"inconsistent return type\n" +
"found : (TwoNumbers|null)\n" +
"required: number");
}
public void testIssue700() throws Exception {
testTypes(
"/**\n" +
" * @param {{text: string}} opt_data\n" +
" * @return {string}\n" +
" */\n" +
"function temp1(opt_data) {\n" +
" return opt_data.text;\n" +
"}\n" +
"\n" +
"/**\n" +
" * @param {{activity: (boolean|number|string|null|Object)}} opt_data\n" +
" * @return {string}\n" +
" */\n" +
"function temp2(opt_data) {\n" +
" /** @notypecheck */\n" +
" function __inner() {\n" +
" return temp1(opt_data.activity);\n" +
" }\n" +
" return __inner();\n" +
"}\n" +
"\n" +
"/**\n" +
" * @param {{n: number, text: string, b: boolean}} opt_data\n" +
" * @return {string}\n" +
" */\n" +
"function temp3(opt_data) {\n" +
" return 'n: ' + opt_data.n + ', t: ' + opt_data.text + '.';\n" +
"}\n" +
"\n" +
"function callee() {\n" +
" var output = temp3({\n" +
" n: 0,\n" +
" text: 'a string',\n" +
" b: true\n" +
" })\n" +
" alert(output);\n" +
"}\n" +
"\n" +
"callee();");
}
public void testIssue725() throws Exception {
testTypes(
"/** @typedef {{name: string}} */ var RecordType1;" +
"/** @typedef {{name2: string}} */ var RecordType2;" +
"/** @param {RecordType1} rec */ function f(rec) {" +
" alert(rec.name2);" +
"}",
"Property name2 never defined on rec");
}
public void testIssue726() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @param {number} x */ Foo.prototype.bar = function(x) {};" +
"/** @return {!Function} */ " +
"Foo.prototype.getDeferredBar = function() { " +
" var self = this;" +
" return function() {" +
" self.bar(true);" +
" };" +
"};",
"actual parameter 1 of Foo.prototype.bar does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testIssue765() throws Exception {
testTypes(
"/** @constructor */" +
"var AnotherType = function (parent) {" +
" /** @param {string} stringParameter Description... */" +
" this.doSomething = function (stringParameter) {};" +
"};" +
"/** @constructor */" +
"var YetAnotherType = function () {" +
" this.field = new AnotherType(self);" +
" this.testfun=function(stringdata) {" +
" this.field.doSomething(null);" +
" };" +
"};",
"actual parameter 1 of AnotherType.doSomething " +
"does not match formal parameter\n" +
"found : null\n" +
"required: string");
}
public void testIssue783() throws Exception {
testTypes(
"/** @constructor */" +
"var Type = function () {" +
" /** @type {Type} */" +
" this.me_ = this;" +
"};" +
"Type.prototype.doIt = function() {" +
" var me = this.me_;" +
" for (var i = 0; i < me.unknownProp; i++) {}" +
"};",
"Property unknownProp never defined on Type");
}
public void testIssue791() throws Exception {
testTypes(
"/** @param {{func: function()}} obj */" +
"function test1(obj) {}" +
"var fnStruc1 = {};" +
"fnStruc1.func = function() {};" +
"test1(fnStruc1);");
}
public void testIssue810() throws Exception {
testTypes(
"/** @constructor */" +
"var Type = function () {" +
"};" +
"Type.prototype.doIt = function(obj) {" +
" this.prop = obj.unknownProp;" +
"};",
"Property unknownProp never defined on obj");
}
/**
* Tests that the || operator is type checked correctly, that is of
* the type of the first argument or of the second argument. See
* bugid 592170 for more details.
*/
public void testBug592170() throws Exception {
testTypes(
"/** @param {Function} opt_f ... */" +
"function foo(opt_f) {" +
" /** @type {Function} */" +
" return opt_f || function () {};" +
"}",
"Type annotations are not allowed here. Are you missing parentheses?");
}
/**
* Tests that undefined can be compared shallowly to a value of type
* (number,undefined) regardless of the side on which the undefined
* value is.
*/
public void testBug901455() throws Exception {
testTypes("/** @return {(number,undefined)} */ function a() { return 3; }" +
"var b = undefined === a()");
testTypes("/** @return {(number,undefined)} */ function a() { return 3; }" +
"var b = a() === undefined");
}
/**
* Tests that the match method of strings returns nullable arrays.
*/
public void testBug908701() throws Exception {
testTypes("/** @type {String} */var s = new String('foo');" +
"var b = s.match(/a/) != null;");
}
/**
* Tests that named types play nicely with subtyping.
*/
public void testBug908625() throws Exception {
testTypes("/** @constructor */function A(){}" +
"/** @constructor\n * @extends A */function B(){}" +
"/** @param {B} b" +
"\n @return {(A,undefined)} */function foo(b){return b}");
}
/**
* Tests that assigning two untyped functions to a variable whose type is
* inferred and calling this variable is legal.
*/
public void testBug911118() throws Exception {
// verifying the type assigned to function expressions assigned variables
Scope s = parseAndTypeCheckWithScope("var a = function(){};").scope;
JSType type = s.getVar("a").getType();
assertEquals("function (): undefined", type.toString());
// verifying the bug example
testTypes("function nullFunction() {};" +
"var foo = nullFunction;" +
"foo = function() {};" +
"foo();");
}
public void testBug909000() throws Exception {
testTypes("/** @constructor */function A(){}\n" +
"/** @param {!A} a\n" +
"@return {boolean}*/\n" +
"function y(a) { return a }",
"inconsistent return type\n" +
"found : A\n" +
"required: boolean");
}
public void testBug930117() throws Exception {
testTypes(
"/** @param {boolean} x */function f(x){}" +
"f(null);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : null\n" +
"required: boolean");
}
public void testBug1484445() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @type {number?} */ Foo.prototype.bar = null;" +
"/** @type {number?} */ Foo.prototype.baz = null;" +
"/** @param {Foo} foo */" +
"function f(foo) {" +
" while (true) {" +
" if (foo.bar == null && foo.baz == null) {" +
" foo.bar;" +
" }" +
" }" +
"}");
}
public void testBug1859535() throws Exception {
testTypes(
"/**\n" +
" * @param {Function} childCtor Child class.\n" +
" * @param {Function} parentCtor Parent class.\n" +
" */" +
"var inherits = function(childCtor, parentCtor) {" +
" /** @constructor */" +
" function tempCtor() {};" +
" tempCtor.prototype = parentCtor.prototype;" +
" childCtor.superClass_ = parentCtor.prototype;" +
" childCtor.prototype = new tempCtor();" +
" /** @override */ childCtor.prototype.constructor = childCtor;" +
"};" +
"/**" +
" * @param {Function} constructor\n" +
" * @param {Object} var_args\n" +
" * @return {Object}\n" +
" */" +
"var factory = function(constructor, var_args) {" +
" /** @constructor */" +
" var tempCtor = function() {};" +
" tempCtor.prototype = constructor.prototype;" +
" var obj = new tempCtor();" +
" constructor.apply(obj, arguments);" +
" return obj;" +
"};");
}
public void testBug1940591() throws Exception {
testTypes(
"/** @type {Object} */" +
"var a = {};\n" +
"/** @type {number} */\n" +
"a.name = 0;\n" +
"/**\n" +
" * @param {Function} x anything.\n" +
" */\n" +
"a.g = function(x) { x.name = 'a'; }");
}
public void testBug1942972() throws Exception {
testTypes(
"var google = {\n" +
" gears: {\n" +
" factory: {},\n" +
" workerPool: {}\n" +
" }\n" +
"};\n" +
"\n" +
"google.gears = {factory: {}};\n");
}
public void testBug1943776() throws Exception {
testTypes(
"/** @return {{foo: Array}} */" +
"function bar() {" +
" return {foo: []};" +
"}");
}
public void testBug1987544() throws Exception {
testTypes(
"/** @param {string} x */ function foo(x) {}" +
"var duration;" +
"if (true && !(duration = 3)) {" +
" foo(duration);" +
"}",
"actual parameter 1 of foo does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testBug1940769() throws Exception {
testTypes(
"/** @return {!Object} */ " +
"function proto(obj) { return obj.prototype; }" +
"/** @constructor */ function Map() {}" +
"/**\n" +
" * @constructor\n" +
" * @extends {Map}\n" +
" */" +
"function Map2() { Map.call(this); };" +
"Map2.prototype = proto(Map);");
}
public void testBug2335992() throws Exception {
testTypes(
"/** @return {*} */ function f() { return 3; }" +
"var x = f();" +
"/** @type {string} */" +
"x.y = 3;",
"assignment\n" +
"found : number\n" +
"required: string");
}
public void testBug2341812() throws Exception {
testTypes(
"/** @interface */" +
"function EventTarget() {}" +
"/** @constructor \n * @implements {EventTarget} */" +
"function Node() {}" +
"/** @type {number} */ Node.prototype.index;" +
"/** @param {EventTarget} x \n * @return {string} */" +
"function foo(x) { return x.index; }");
}
public void testBug7701884() throws Exception {
testTypes(
"/**\n" +
" * @param {Array.<T>} x\n" +
" * @param {function(T)} y\n" +
" * @template T\n" +
" */\n" +
"var forEach = function(x, y) {\n" +
" for (var i = 0; i < x.length; i++) y(x[i]);\n" +
"};" +
"/** @param {number} x */" +
"function f(x) {}" +
"/** @param {?} x */" +
"function h(x) {" +
" var top = null;" +
" forEach(x, function(z) { top = z; });" +
" if (top) f(top);" +
"}");
}
public void testScopedConstructors1() throws Exception {
testTypes(
"function foo1() { " +
" /** @constructor */ function Bar() { " +
" /** @type {number} */ this.x = 3;" +
" }" +
"}" +
"function foo2() { " +
" /** @constructor */ function Bar() { " +
" /** @type {string} */ this.x = 'y';" +
" }" +
" /** " +
" * @param {Bar} b\n" +
" * @return {number}\n" +
" */" +
" function baz(b) { return b.x; }" +
"}",
"inconsistent return type\n" +
"found : string\n" +
"required: number");
}
public void testScopedConstructors2() throws Exception {
testTypes(
"/** @param {Function} f */" +
"function foo1(f) {" +
" /** @param {Function} g */" +
" f.prototype.bar = function(g) {};" +
"}");
}
public void testQualifiedNameInference1() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @type {number?} */ Foo.prototype.bar = null;" +
"/** @type {number?} */ Foo.prototype.baz = null;" +
"/** @param {Foo} foo */" +
"function f(foo) {" +
" while (true) {" +
" if (!foo.baz) break; " +
" foo.bar = null;" +
" }" +
// Tests a bug where this condition always evaluated to true.
" return foo.bar == null;" +
"}");
}
public void testQualifiedNameInference2() throws Exception {
testTypes(
"var x = {};" +
"x.y = c;" +
"function f(a, b) {" +
" if (a) {" +
" if (b) " +
" x.y = 2;" +
" else " +
" x.y = 1;" +
" }" +
" return x.y == null;" +
"}");
}
public void testQualifiedNameInference3() throws Exception {
testTypes(
"var x = {};" +
"x.y = c;" +
"function f(a, b) {" +
" if (a) {" +
" if (b) " +
" x.y = 2;" +
" else " +
" x.y = 1;" +
" }" +
" return x.y == null;" +
"} function g() { x.y = null; }");
}
public void testQualifiedNameInference4() throws Exception {
testTypes(
"/** @param {string} x */ function f(x) {}\n" +
"/**\n" +
" * @param {?string} x \n" +
" * @constructor\n" +
" */" +
"function Foo(x) { this.x_ = x; }\n" +
"Foo.prototype.bar = function() {" +
" if (this.x_) { f(this.x_); }" +
"};");
}
public void testQualifiedNameInference5() throws Exception {
testTypes(
"var ns = {}; " +
"(function() { " +
" /** @param {number} x */ ns.foo = function(x) {}; })();" +
"(function() { ns.foo(true); })();",
"actual parameter 1 of ns.foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testQualifiedNameInference6() throws Exception {
testTypes(
"/** @const */ var ns = {}; " +
"/** @param {number} x */ ns.foo = function(x) {};" +
"(function() { " +
" ns.foo = function(x) {};" +
" ns.foo(true); " +
"})();",
"actual parameter 1 of ns.foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testQualifiedNameInference7() throws Exception {
testTypes(
"var ns = {}; " +
"(function() { " +
" /** @constructor \n * @param {number} x */ " +
" ns.Foo = function(x) {};" +
" /** @param {ns.Foo} x */ function f(x) {}" +
" f(new ns.Foo(true));" +
"})();",
"actual parameter 1 of ns.Foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testQualifiedNameInference8() throws Exception {
// We may need to reshuffle name resolution order so that the @param
// type resolves correctly.
testClosureTypesMultipleWarnings(
"var ns = {}; " +
"(function() { " +
" /** @constructor \n * @param {number} x */ " +
" ns.Foo = function(x) {};" +
"})();" +
"/** @param {ns.Foo} x */ function f(x) {}" +
"f(new ns.Foo(true));",
Lists.newArrayList(
"Bad type annotation. Unknown type ns.Foo",
"actual parameter 1 of ns.Foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number"));
}
public void testQualifiedNameInference9() throws Exception {
testTypes(
"var ns = {}; " +
"ns.ns2 = {}; " +
"(function() { " +
" /** @constructor \n * @param {number} x */ " +
" ns.ns2.Foo = function(x) {};" +
" /** @param {ns.ns2.Foo} x */ function f(x) {}" +
" f(new ns.ns2.Foo(true));" +
"})();",
"actual parameter 1 of ns.ns2.Foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testQualifiedNameInference10() throws Exception {
testTypes(
"var ns = {}; " +
"ns.ns2 = {}; " +
"(function() { " +
" /** @interface */ " +
" ns.ns2.Foo = function() {};" +
" /** @constructor \n * @implements {ns.ns2.Foo} */ " +
" function F() {}" +
" (new F());" +
"})();");
}
public void testQualifiedNameInference11() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"function f() {" +
" var x = new Foo();" +
" x.onload = function() {" +
" x.onload = null;" +
" };" +
"}");
}
public void testQualifiedNameInference12() throws Exception {
// We should be able to tell that the two 'this' properties
// are different.
testTypes(
"/** @param {function(this:Object)} x */ function f(x) {}" +
"/** @constructor */ function Foo() {" +
" /** @type {number} */ this.bar = 3;" +
" f(function() { this.bar = true; });" +
"}");
}
public void testQualifiedNameInference13() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"function f(z) {" +
" var x = new Foo();" +
" if (z) {" +
" x.onload = function() {};" +
" } else {" +
" x.onload = null;" +
" };" +
"}");
}
public void testSheqRefinedScope() throws Exception {
Node n = parseAndTypeCheck(
"/** @constructor */function A() {}\n" +
"/** @constructor \n @extends A */ function B() {}\n" +
"/** @return {number} */\n" +
"B.prototype.p = function() { return 1; }\n" +
"/** @param {A} a\n @param {B} b */\n" +
"function f(a, b) {\n" +
" b.p();\n" +
" if (a === b) {\n" +
" b.p();\n" +
" }\n" +
"}");
Node nodeC = n.getLastChild().getLastChild().getLastChild().getLastChild()
.getLastChild().getLastChild();
JSType typeC = nodeC.getJSType();
assertTrue(typeC.isNumber());
Node nodeB = nodeC.getFirstChild().getFirstChild();
JSType typeB = nodeB.getJSType();
assertEquals("B", typeB.toString());
}
public void testAssignToUntypedVariable() throws Exception {
Node n = parseAndTypeCheck("var z; z = 1;");
Node assign = n.getLastChild().getFirstChild();
Node node = assign.getFirstChild();
assertFalse(node.getJSType().isUnknownType());
assertEquals("number", node.getJSType().toString());
}
public void testAssignToUntypedProperty() throws Exception {
Node n = parseAndTypeCheck(
"/** @constructor */ function Foo() {}\n" +
"Foo.prototype.a = 1;" +
"(new Foo).a;");
Node node = n.getLastChild().getFirstChild();
assertFalse(node.getJSType().isUnknownType());
assertTrue(node.getJSType().isNumber());
}
public void testNew1() throws Exception {
testTypes("new 4", TypeCheck.NOT_A_CONSTRUCTOR);
}
public void testNew2() throws Exception {
testTypes("var Math = {}; new Math()", TypeCheck.NOT_A_CONSTRUCTOR);
}
public void testNew3() throws Exception {
testTypes("new Date()");
}
public void testNew4() throws Exception {
testTypes("/** @constructor */function A(){}; new A();");
}
public void testNew5() throws Exception {
testTypes("function A(){}; new A();", TypeCheck.NOT_A_CONSTRUCTOR);
}
public void testNew6() throws Exception {
TypeCheckResult p =
parseAndTypeCheckWithScope("/** @constructor */function A(){};" +
"var a = new A();");
JSType aType = p.scope.getVar("a").getType();
assertTrue(aType instanceof ObjectType);
ObjectType aObjectType = (ObjectType) aType;
assertEquals("A", aObjectType.getConstructor().getReferenceName());
}
public void testNew7() throws Exception {
testTypes("/** @param {Function} opt_constructor */" +
"function foo(opt_constructor) {" +
"if (opt_constructor) { new opt_constructor; }" +
"}");
}
public void testNew8() throws Exception {
testTypes("/** @param {Function} opt_constructor */" +
"function foo(opt_constructor) {" +
"new opt_constructor;" +
"}");
}
public void testNew9() throws Exception {
testTypes("/** @param {Function} opt_constructor */" +
"function foo(opt_constructor) {" +
"new (opt_constructor || Array);" +
"}");
}
public void testNew10() throws Exception {
testTypes("var goog = {};" +
"/** @param {Function} opt_constructor */" +
"goog.Foo = function (opt_constructor) {" +
"new (opt_constructor || Array);" +
"}");
}
public void testNew11() throws Exception {
testTypes("/** @param {Function} c1 */" +
"function f(c1) {" +
" var c2 = function(){};" +
" c1.prototype = new c2;" +
"}", TypeCheck.NOT_A_CONSTRUCTOR);
}
public void testNew12() throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope("var a = new Array();");
Var a = p.scope.getVar("a");
assertTypeEquals(ARRAY_TYPE, a.getType());
}
public void testNew13() throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope(
"/** @constructor */function FooBar(){};" +
"var a = new FooBar();");
Var a = p.scope.getVar("a");
assertTrue(a.getType() instanceof ObjectType);
assertEquals("FooBar", a.getType().toString());
}
public void testNew14() throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope(
"/** @constructor */var FooBar = function(){};" +
"var a = new FooBar();");
Var a = p.scope.getVar("a");
assertTrue(a.getType() instanceof ObjectType);
assertEquals("FooBar", a.getType().toString());
}
public void testNew15() throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope(
"var goog = {};" +
"/** @constructor */goog.A = function(){};" +
"var a = new goog.A();");
Var a = p.scope.getVar("a");
assertTrue(a.getType() instanceof ObjectType);
assertEquals("goog.A", a.getType().toString());
}
public void testNew16() throws Exception {
testTypes(
"/** \n" +
" * @param {string} x \n" +
" * @constructor \n" +
" */" +
"function Foo(x) {}" +
"function g() { new Foo(1); }",
"actual parameter 1 of Foo does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testNew17() throws Exception {
testTypes("var goog = {}; goog.x = 3; new goog.x",
"cannot instantiate non-constructor");
}
public void testNew18() throws Exception {
testTypes("var goog = {};" +
"/** @constructor */ goog.F = function() {};" +
"/** @constructor */ goog.G = goog.F;");
}
public void testName1() throws Exception {
assertTypeEquals(VOID_TYPE, testNameNode("undefined"));
}
public void testName2() throws Exception {
assertTypeEquals(OBJECT_FUNCTION_TYPE, testNameNode("Object"));
}
public void testName3() throws Exception {
assertTypeEquals(ARRAY_FUNCTION_TYPE, testNameNode("Array"));
}
public void testName4() throws Exception {
assertTypeEquals(DATE_FUNCTION_TYPE, testNameNode("Date"));
}
public void testName5() throws Exception {
assertTypeEquals(REGEXP_FUNCTION_TYPE, testNameNode("RegExp"));
}
/**
* Type checks a NAME node and retrieve its type.
*/
private JSType testNameNode(String name) {
Node node = Node.newString(Token.NAME, name);
Node parent = new Node(Token.SCRIPT, node);
parent.setInputId(new InputId("code"));
Node externs = new Node(Token.SCRIPT);
externs.setInputId(new InputId("externs"));
Node externAndJsRoot = new Node(Token.BLOCK, externs, parent);
externAndJsRoot.setIsSyntheticBlock(true);
makeTypeCheck().processForTesting(null, parent);
return node.getJSType();
}
public void testBitOperation1() throws Exception {
testTypes("/**@return {void}*/function foo(){ ~foo(); }",
"operator ~ cannot be applied to undefined");
}
public void testBitOperation2() throws Exception {
testTypes("/**@return {void}*/function foo(){var a = foo()<<3;}",
"operator << cannot be applied to undefined");
}
public void testBitOperation3() throws Exception {
testTypes("/**@return {void}*/function foo(){var a = 3<<foo();}",
"operator << cannot be applied to undefined");
}
public void testBitOperation4() throws Exception {
testTypes("/**@return {void}*/function foo(){var a = foo()>>>3;}",
"operator >>> cannot be applied to undefined");
}
public void testBitOperation5() throws Exception {
testTypes("/**@return {void}*/function foo(){var a = 3>>>foo();}",
"operator >>> cannot be applied to undefined");
}
public void testBitOperation6() throws Exception {
testTypes("/**@return {!Object}*/function foo(){var a = foo()&3;}",
"bad left operand to bitwise operator\n" +
"found : Object\n" +
"required: (boolean|null|number|string|undefined)");
}
public void testBitOperation7() throws Exception {
testTypes("var x = null; x |= undefined; x &= 3; x ^= '3'; x |= true;");
}
public void testBitOperation8() throws Exception {
testTypes("var x = void 0; x |= new Number(3);");
}
public void testBitOperation9() throws Exception {
testTypes("var x = void 0; x |= {};",
"bad right operand to bitwise operator\n" +
"found : {}\n" +
"required: (boolean|null|number|string|undefined)");
}
public void testCall1() throws Exception {
testTypes("3();", "number expressions are not callable");
}
public void testCall2() throws Exception {
testTypes("/** @param {!Number} foo*/function bar(foo){ bar('abc'); }",
"actual parameter 1 of bar does not match formal parameter\n" +
"found : string\n" +
"required: Number");
}
public void testCall3() throws Exception {
// We are checking that an unresolved named type can successfully
// meet with a functional type to produce a callable type.
testTypes("/** @type {Function|undefined} */var opt_f;" +
"/** @type {some.unknown.type} */var f1;" +
"var f2 = opt_f || f1;" +
"f2();",
"Bad type annotation. Unknown type some.unknown.type");
}
public void testCall4() throws Exception {
testTypes("/**@param {!RegExp} a*/var foo = function bar(a){ bar('abc'); }",
"actual parameter 1 of bar does not match formal parameter\n" +
"found : string\n" +
"required: RegExp");
}
public void testCall5() throws Exception {
testTypes("/**@param {!RegExp} a*/var foo = function bar(a){ foo('abc'); }",
"actual parameter 1 of foo does not match formal parameter\n" +
"found : string\n" +
"required: RegExp");
}
public void testCall6() throws Exception {
testTypes("/** @param {!Number} foo*/function bar(foo){}" +
"bar('abc');",
"actual parameter 1 of bar does not match formal parameter\n" +
"found : string\n" +
"required: Number");
}
public void testCall7() throws Exception {
testTypes("/** @param {!RegExp} a*/var foo = function bar(a){};" +
"foo('abc');",
"actual parameter 1 of foo does not match formal parameter\n" +
"found : string\n" +
"required: RegExp");
}
public void testCall8() throws Exception {
testTypes("/** @type {Function|number} */var f;f();",
"(Function|number) expressions are " +
"not callable");
}
public void testCall9() throws Exception {
testTypes(
"var goog = {};" +
"/** @constructor */ goog.Foo = function() {};" +
"/** @param {!goog.Foo} a */ var bar = function(a){};" +
"bar('abc');",
"actual parameter 1 of bar does not match formal parameter\n" +
"found : string\n" +
"required: goog.Foo");
}
public void testCall10() throws Exception {
testTypes("/** @type {Function} */var f;f();");
}
public void testCall11() throws Exception {
testTypes("var f = new Function(); f();");
}
public void testFunctionCall1() throws Exception {
testTypes(
"/** @param {number} x */ var foo = function(x) {};" +
"foo.call(null, 3);");
}
public void testFunctionCall2() throws Exception {
testTypes(
"/** @param {number} x */ var foo = function(x) {};" +
"foo.call(null, 'bar');",
"actual parameter 2 of foo.call does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testFunctionCall3() throws Exception {
testTypes(
"/** @param {number} x \n * @constructor */ " +
"var Foo = function(x) { this.bar.call(null, x); };" +
"/** @type {function(number)} */ Foo.prototype.bar;");
}
public void testFunctionCall4() throws Exception {
testTypes(
"/** @param {string} x \n * @constructor */ " +
"var Foo = function(x) { this.bar.call(null, x); };" +
"/** @type {function(number)} */ Foo.prototype.bar;",
"actual parameter 2 of this.bar.call " +
"does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testFunctionCall5() throws Exception {
testTypes(
"/** @param {Function} handler \n * @constructor */ " +
"var Foo = function(handler) { handler.call(this, x); };");
}
public void testFunctionCall6() throws Exception {
testTypes(
"/** @param {Function} handler \n * @constructor */ " +
"var Foo = function(handler) { handler.apply(this, x); };");
}
public void testFunctionCall7() throws Exception {
testTypes(
"/** @param {Function} handler \n * @param {Object} opt_context */ " +
"var Foo = function(handler, opt_context) { " +
" handler.call(opt_context, x);" +
"};");
}
public void testFunctionCall8() throws Exception {
testTypes(
"/** @param {Function} handler \n * @param {Object} opt_context */ " +
"var Foo = function(handler, opt_context) { " +
" handler.apply(opt_context, x);" +
"};");
}
public void testFunctionBind1() throws Exception {
testTypes(
"/** @type {function(string, number): boolean} */" +
"function f(x, y) { return true; }" +
"f.bind(null, 3);",
"actual parameter 2 of f.bind does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testFunctionBind2() throws Exception {
testTypes(
"/** @type {function(number): boolean} */" +
"function f(x) { return true; }" +
"f(f.bind(null, 3)());",
"actual parameter 1 of f does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testFunctionBind3() throws Exception {
testTypes(
"/** @type {function(number, string): boolean} */" +
"function f(x, y) { return true; }" +
"f.bind(null, 3)(true);",
"actual parameter 1 of function does not match formal parameter\n" +
"found : boolean\n" +
"required: string");
}
public void testFunctionBind4() throws Exception {
testTypes(
"/** @param {...number} x */" +
"function f(x) {}" +
"f.bind(null, 3, 3, 3)(true);",
"actual parameter 1 of function does not match formal parameter\n" +
"found : boolean\n" +
"required: (number|undefined)");
}
public void testFunctionBind5() throws Exception {
testTypes(
"/** @param {...number} x */" +
"function f(x) {}" +
"f.bind(null, true)(3, 3, 3);",
"actual parameter 2 of f.bind does not match formal parameter\n" +
"found : boolean\n" +
"required: (number|undefined)");
}
public void testGoogBind1() throws Exception {
testClosureTypes(
"var goog = {}; goog.bind = function(var_args) {};" +
"/** @type {function(number): boolean} */" +
"function f(x, y) { return true; }" +
"f(goog.bind(f, null, 'x')());",
"actual parameter 1 of f does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testGoogBind2() throws Exception {
// TODO(nicksantos): We do not currently type-check the arguments
// of the goog.bind.
testClosureTypes(
"var goog = {}; goog.bind = function(var_args) {};" +
"/** @type {function(boolean): boolean} */" +
"function f(x, y) { return true; }" +
"f(goog.bind(f, null, 'x')());",
null);
}
public void testCast2() throws Exception {
// can upcast to a base type.
testTypes("/** @constructor */function base() {}\n" +
"/** @constructor\n @extends {base} */function derived() {}\n" +
"/** @type {base} */ var baz = new derived();\n");
}
public void testCast3() throws Exception {
// cannot downcast
testTypes("/** @constructor */function base() {}\n" +
"/** @constructor @extends {base} */function derived() {}\n" +
"/** @type {!derived} */ var baz = new base();\n",
"initializing variable\n" +
"found : base\n" +
"required: derived");
}
public void testCast3a() throws Exception {
// cannot downcast
testTypes("/** @constructor */function Base() {}\n" +
"/** @constructor @extends {Base} */function Derived() {}\n" +
"var baseInstance = new Base();" +
"/** @type {!Derived} */ var baz = baseInstance;\n",
"initializing variable\n" +
"found : Base\n" +
"required: Derived");
}
public void testCast4() throws Exception {
// downcast must be explicit
testTypes("/** @constructor */function base() {}\n" +
"/** @constructor\n * @extends {base} */function derived() {}\n" +
"/** @type {!derived} */ var baz = " +
"/** @type {!derived} */(new base());\n");
}
public void testCast5() throws Exception {
// cannot explicitly cast to an unrelated type
testTypes("/** @constructor */function foo() {}\n" +
"/** @constructor */function bar() {}\n" +
"var baz = /** @type {!foo} */(new bar);\n",
"invalid cast - must be a subtype or supertype\n" +
"from: bar\n" +
"to : foo");
}
public void testCast5a() throws Exception {
// cannot explicitly cast to an unrelated type
testTypes("/** @constructor */function foo() {}\n" +
"/** @constructor */function bar() {}\n" +
"var barInstance = new bar;\n" +
"var baz = /** @type {!foo} */(barInstance);\n",
"invalid cast - must be a subtype or supertype\n" +
"from: bar\n" +
"to : foo");
}
public void testCast6() throws Exception {
// can explicitly cast to a subtype or supertype
testTypes("/** @constructor */function foo() {}\n" +
"/** @constructor \n @extends foo */function bar() {}\n" +
"var baz = /** @type {!bar} */(new bar);\n" +
"var baz = /** @type {!foo} */(new foo);\n" +
"var baz = /** @type {bar} */(new bar);\n" +
"var baz = /** @type {foo} */(new foo);\n" +
"var baz = /** @type {!foo} */(new bar);\n" +
"var baz = /** @type {!bar} */(new foo);\n" +
"var baz = /** @type {foo} */(new bar);\n" +
"var baz = /** @type {bar} */(new foo);\n");
}
public void testCast7() throws Exception {
testTypes("var x = /** @type {foo} */ (new Object());",
"Bad type annotation. Unknown type foo");
}
public void testCast8() throws Exception {
testTypes("function f() { return /** @type {foo} */ (new Object()); }",
"Bad type annotation. Unknown type foo");
}
public void testCast9() throws Exception {
testTypes("var foo = {};" +
"function f() { return /** @type {foo} */ (new Object()); }",
"Bad type annotation. Unknown type foo");
}
public void testCast10() throws Exception {
testTypes("var foo = function() {};" +
"function f() { return /** @type {foo} */ (new Object()); }",
"Bad type annotation. Unknown type foo");
}
public void testCast11() throws Exception {
testTypes("var goog = {}; goog.foo = {};" +
"function f() { return /** @type {goog.foo} */ (new Object()); }",
"Bad type annotation. Unknown type goog.foo");
}
public void testCast12() throws Exception {
testTypes("var goog = {}; goog.foo = function() {};" +
"function f() { return /** @type {goog.foo} */ (new Object()); }",
"Bad type annotation. Unknown type goog.foo");
}
public void testCast13() throws Exception {
// Test to make sure that the forward-declaration still allows for
// a warning.
testClosureTypes("var goog = {}; " +
"goog.addDependency('zzz.js', ['goog.foo'], []);" +
"goog.foo = function() {};" +
"function f() { return /** @type {goog.foo} */ (new Object()); }",
"Bad type annotation. Unknown type goog.foo");
}
public void testCast14() throws Exception {
// Test to make sure that the forward-declaration still prevents
// some warnings.
testClosureTypes("var goog = {}; " +
"goog.addDependency('zzz.js', ['goog.bar'], []);" +
"function f() { return /** @type {goog.bar} */ (new Object()); }",
null);
}
public void testCast15() throws Exception {
// This fixes a bug where a type cast on an object literal
// would cause a run-time cast exception if the node was visited
// more than once.
//
// Some code assumes that an object literal must have a object type,
// while because of the cast, it could have any type (including
// a union).
testTypes(
"for (var i = 0; i < 10; i++) {" +
"var x = /** @type {Object|number} */ ({foo: 3});" +
"/** @param {number} x */ function f(x) {}" +
"f(x.foo);" +
"f([].foo);" +
"}",
"Property foo never defined on Array");
}
public void testCast16() throws Exception {
// A type cast should not invalidate the checks on the members
testTypes(
"for (var i = 0; i < 10; i++) {" +
"var x = /** @type {Object|number} */ (" +
" {/** @type {string} */ foo: 3});" +
"}",
"assignment to property foo of Object\n" +
"found : number\n" +
"required: string");
}
public void testCast17a() throws Exception {
// Mostly verifying that rhino actually understands these JsDocs.
testTypes("/** @constructor */ function Foo() {} \n" +
"/** @type {Foo} */ var x = /** @type {Foo} */ (y)");
testTypes("/** @constructor */ function Foo() {} \n" +
"/** @type {Foo} */ var x = (/** @type {Foo} */ y)");
}
public void testCast17b() throws Exception {
// Mostly verifying that rhino actually understands these JsDocs.
testTypes("/** @constructor */ function Foo() {} \n" +
"/** @type {Foo} */ var x = /** @type {Foo} */ ({})");
}
public void testCast18() throws Exception {
// Mostly verifying that legacy annotations are applied
// despite the parser warning.
testTypes("/** @constructor */ function Foo() {} \n" +
"/** @type {Foo} */ var x = (/** @type {Foo} */ {})",
"Type annotations are not allowed here. " +
"Are you missing parentheses?");
// Not really encourage because of possible ambiguity but it works.
testTypes("/** @constructor */ function Foo() {} \n" +
"/** @type {Foo} */ var x = /** @type {Foo} */ {}",
"Type annotations are not allowed here. " +
"Are you missing parentheses?");
}
public void testCast19() throws Exception {
testTypes(
"var x = 'string';\n" +
"/** @type {number} */\n" +
"var y = /** @type {number} */(x);",
"invalid cast - must be a subtype or supertype\n" +
"from: string\n" +
"to : number");
}
public void testCast20() throws Exception {
testTypes(
"/** @enum {boolean|null} */\n" +
"var X = {" +
" AA: true," +
" BB: false," +
" CC: null" +
"};\n" +
"var y = /** @type {X} */(true);");
}
public void testCast21() throws Exception {
testTypes(
"/** @enum {boolean|null} */\n" +
"var X = {" +
" AA: true," +
" BB: false," +
" CC: null" +
"};\n" +
"var value = true;\n" +
"var y = /** @type {X} */(value);");
}
public void testCast22() throws Exception {
testTypes(
"var x = null;\n" +
"var y = /** @type {number} */(x);",
"invalid cast - must be a subtype or supertype\n" +
"from: null\n" +
"to : number");
}
public void testCast23() throws Exception {
testTypes(
"var x = null;\n" +
"var y = /** @type {Number} */(x);");
}
public void testCast24() throws Exception {
testTypes(
"var x = undefined;\n" +
"var y = /** @type {number} */(x);",
"invalid cast - must be a subtype or supertype\n" +
"from: undefined\n" +
"to : number");
}
public void testCast25() throws Exception {
testTypes(
"var x = undefined;\n" +
"var y = /** @type {number|undefined} */(x);");
}
public void testCast26() throws Exception {
testTypes(
"function fn(dir) {\n" +
" var node = dir ? 1 : 2;\n" +
" fn(/** @type {number} */ (node));\n" +
"}");
}
public void testCast27() throws Exception {
// C doesn't implement I but a subtype might.
testTypes(
"/** @interface */ function I() {}\n" +
"/** @constructor */ function C() {}\n" +
"var x = new C();\n" +
"var y = /** @type {I} */(x);");
}
public void testCast27a() throws Exception {
// C doesn't implement I but a subtype might.
testTypes(
"/** @interface */ function I() {}\n" +
"/** @constructor */ function C() {}\n" +
"/** @type {C} */ var x ;\n" +
"var y = /** @type {I} */(x);");
}
public void testCast28() throws Exception {
// C doesn't implement I but a subtype might.
testTypes(
"/** @interface */ function I() {}\n" +
"/** @constructor */ function C() {}\n" +
"/** @type {!I} */ var x;\n" +
"var y = /** @type {C} */(x);");
}
public void testCast28a() throws Exception {
// C doesn't implement I but a subtype might.
testTypes(
"/** @interface */ function I() {}\n" +
"/** @constructor */ function C() {}\n" +
"/** @type {I} */ var x;\n" +
"var y = /** @type {C} */(x);");
}
public void testCast29a() throws Exception {
// C doesn't implement the record type but a subtype might.
testTypes(
"/** @constructor */ function C() {}\n" +
"var x = new C();\n" +
"var y = /** @type {{remoteJids: Array, sessionId: string}} */(x);");
}
public void testCast29b() throws Exception {
// C doesn't implement the record type but a subtype might.
testTypes(
"/** @constructor */ function C() {}\n" +
"/** @type {C} */ var x;\n" +
"var y = /** @type {{prop1: Array, prop2: string}} */(x);");
}
public void testCast29c() throws Exception {
// C doesn't implement the record type but a subtype might.
testTypes(
"/** @constructor */ function C() {}\n" +
"/** @type {{remoteJids: Array, sessionId: string}} */ var x ;\n" +
"var y = /** @type {C} */(x);");
}
public void testCast30() throws Exception {
// Should be able to cast to a looser return type
testTypes(
"/** @constructor */ function C() {}\n" +
"/** @type {function():string} */ var x ;\n" +
"var y = /** @type {function():?} */(x);");
}
public void testCast31() throws Exception {
// Should be able to cast to a tighter parameter type
testTypes(
"/** @constructor */ function C() {}\n" +
"/** @type {function(*)} */ var x ;\n" +
"var y = /** @type {function(string)} */(x);");
}
public void testCast32() throws Exception {
testTypes(
"/** @constructor */ function C() {}\n" +
"/** @type {Object} */ var x ;\n" +
"var y = /** @type {null|{length:number}} */(x);");
}
public void testCast33() throws Exception {
// null and void should be assignable to any type that accepts one or the
// other or both.
testTypes(
"/** @constructor */ function C() {}\n" +
"/** @type {null|undefined} */ var x ;\n" +
"var y = /** @type {string?|undefined} */(x);");
testTypes(
"/** @constructor */ function C() {}\n" +
"/** @type {null|undefined} */ var x ;\n" +
"var y = /** @type {string|undefined} */(x);");
testTypes(
"/** @constructor */ function C() {}\n" +
"/** @type {null|undefined} */ var x ;\n" +
"var y = /** @type {string?} */(x);");
testTypes(
"/** @constructor */ function C() {}\n" +
"/** @type {null|undefined} */ var x ;\n" +
"var y = /** @type {null} */(x);");
}
public void testCast34a() throws Exception {
testTypes(
"/** @constructor */ function C() {}\n" +
"/** @type {Object} */ var x ;\n" +
"var y = /** @type {Function} */(x);");
}
public void testCast34b() throws Exception {
testTypes(
"/** @constructor */ function C() {}\n" +
"/** @type {Function} */ var x ;\n" +
"var y = /** @type {Object} */(x);");
}
public void testNestedCasts() throws Exception {
testTypes("/** @constructor */var T = function() {};\n" +
"/** @constructor */var V = function() {};\n" +
"/**\n" +
"* @param {boolean} b\n" +
"* @return {T|V}\n" +
"*/\n" +
"function f(b) { return b ? new T() : new V(); }\n" +
"/**\n" +
"* @param {boolean} b\n" +
"* @return {boolean|undefined}\n" +
"*/\n" +
"function g(b) { return b ? true : undefined; }\n" +
"/** @return {T} */\n" +
"function h() {\n" +
"return /** @type {T} */ (f(/** @type {boolean} */ (g(true))));\n" +
"}");
}
public void testNativeCast1() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"f(String(true));",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testNativeCast2() throws Exception {
testTypes(
"/** @param {string} x */ function f(x) {}" +
"f(Number(true));",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testNativeCast3() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"f(Boolean(''));",
"actual parameter 1 of f does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testNativeCast4() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"f(Error(''));",
"actual parameter 1 of f does not match formal parameter\n" +
"found : Error\n" +
"required: number");
}
public void testBadConstructorCall() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo();",
"Constructor function (new:Foo): undefined should be called " +
"with the \"new\" keyword");
}
public void testTypeof() throws Exception {
testTypes("/**@return {void}*/function foo(){ var a = typeof foo(); }");
}
public void testTypeof2() throws Exception {
testTypes("function f(){ if (typeof 123 == 'numbr') return 321; }",
"unknown type: numbr");
}
public void testTypeof3() throws Exception {
testTypes("function f() {" +
"return (typeof 123 == 'number' ||" +
"typeof 123 == 'string' ||" +
"typeof 123 == 'boolean' ||" +
"typeof 123 == 'undefined' ||" +
"typeof 123 == 'function' ||" +
"typeof 123 == 'object' ||" +
"typeof 123 == 'unknown'); }");
}
public void testConstructorType1() throws Exception {
testTypes("/**@constructor*/function Foo(){}" +
"/**@type{!Foo}*/var f = new Date();",
"initializing variable\n" +
"found : Date\n" +
"required: Foo");
}
public void testConstructorType2() throws Exception {
testTypes("/**@constructor*/function Foo(){\n" +
"/**@type{Number}*/this.bar = new Number(5);\n" +
"}\n" +
"/**@type{Foo}*/var f = new Foo();\n" +
"/**@type{Number}*/var n = f.bar;");
}
public void testConstructorType3() throws Exception {
// Reverse the declaration order so that we know that Foo is getting set
// even on an out-of-order declaration sequence.
testTypes("/**@type{Foo}*/var f = new Foo();\n" +
"/**@type{Number}*/var n = f.bar;" +
"/**@constructor*/function Foo(){\n" +
"/**@type{Number}*/this.bar = new Number(5);\n" +
"}\n");
}
public void testConstructorType4() throws Exception {
testTypes("/**@constructor*/function Foo(){\n" +
"/**@type{!Number}*/this.bar = new Number(5);\n" +
"}\n" +
"/**@type{!Foo}*/var f = new Foo();\n" +
"/**@type{!String}*/var n = f.bar;",
"initializing variable\n" +
"found : Number\n" +
"required: String");
}
public void testConstructorType5() throws Exception {
testTypes("/**@constructor*/function Foo(){}\n" +
"if (Foo){}\n");
}
public void testConstructorType6() throws Exception {
testTypes("/** @constructor */\n" +
"function bar() {}\n" +
"function _foo() {\n" +
" /** @param {bar} x */\n" +
" function f(x) {}\n" +
"}");
}
public void testConstructorType7() throws Exception {
TypeCheckResult p =
parseAndTypeCheckWithScope("/** @constructor */function A(){};");
JSType type = p.scope.getVar("A").getType();
assertTrue(type instanceof FunctionType);
FunctionType fType = (FunctionType) type;
assertEquals("A", fType.getReferenceName());
}
public void testConstructorType8() throws Exception {
testTypes(
"var ns = {};" +
"ns.create = function() { return function() {}; };" +
"/** @constructor */ ns.Foo = ns.create();" +
"ns.Foo.prototype = {x: 0, y: 0};" +
"/**\n" +
" * @param {ns.Foo} foo\n" +
" * @return {string}\n" +
" */\n" +
"function f(foo) {" +
" return foo.x;" +
"}",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testConstructorType9() throws Exception {
testTypes(
"var ns = {};" +
"ns.create = function() { return function() {}; };" +
"ns.extend = function(x) { return x; };" +
"/** @constructor */ ns.Foo = ns.create();" +
"ns.Foo.prototype = ns.extend({x: 0, y: 0});" +
"/**\n" +
" * @param {ns.Foo} foo\n" +
" * @return {string}\n" +
" */\n" +
"function f(foo) {" +
" return foo.x;" +
"}");
}
public void testConstructorType10() throws Exception {
testTypes("/** @constructor */" +
"function NonStr() {}" +
"/**\n" +
" * @constructor\n" +
" * @struct\n" +
" * @extends{NonStr}\n" +
" */" +
"function NonStrKid() {}",
"NonStrKid cannot extend this type; " +
"structs can only extend structs");
}
public void testConstructorType11() throws Exception {
testTypes("/** @constructor */" +
"function NonDict() {}" +
"/**\n" +
" * @constructor\n" +
" * @dict\n" +
" * @extends{NonDict}\n" +
" */" +
"function NonDictKid() {}",
"NonDictKid cannot extend this type; " +
"dicts can only extend dicts");
}
public void testConstructorType12() throws Exception {
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */\n" +
"function Bar() {}\n" +
"Bar.prototype = {};\n",
"Bar cannot extend this type; " +
"structs can only extend structs");
}
public void testBadStruct() throws Exception {
testTypes("/** @struct */function Struct1() {}",
"@struct used without @constructor for Struct1");
}
public void testBadDict() throws Exception {
testTypes("/** @dict */function Dict1() {}",
"@dict used without @constructor for Dict1");
}
public void testAnonymousPrototype1() throws Exception {
testTypes(
"var ns = {};" +
"/** @constructor */ ns.Foo = function() {" +
" this.bar(3, 5);" +
"};" +
"ns.Foo.prototype = {" +
" bar: function(x) {}" +
"};",
"Function ns.Foo.prototype.bar: called with 2 argument(s). " +
"Function requires at least 1 argument(s) and no more " +
"than 1 argument(s).");
}
public void testAnonymousPrototype2() throws Exception {
testTypes(
"/** @interface */ var Foo = function() {};" +
"Foo.prototype = {" +
" foo: function(x) {}" +
"};" +
"/**\n" +
" * @constructor\n" +
" * @implements {Foo}\n" +
" */ var Bar = function() {};",
"property foo on interface Foo is not implemented by type Bar");
}
public void testAnonymousType1() throws Exception {
testTypes("function f() { return {}; }" +
"/** @constructor */\n" +
"f().bar = function() {};");
}
public void testAnonymousType2() throws Exception {
testTypes("function f() { return {}; }" +
"/** @interface */\n" +
"f().bar = function() {};");
}
public void testAnonymousType3() throws Exception {
testTypes("function f() { return {}; }" +
"/** @enum */\n" +
"f().bar = {FOO: 1};");
}
public void testBang1() throws Exception {
testTypes("/** @param {Object} x\n@return {!Object} */\n" +
"function f(x) { return x; }",
"inconsistent return type\n" +
"found : (Object|null)\n" +
"required: Object");
}
public void testBang2() throws Exception {
testTypes("/** @param {Object} x\n@return {!Object} */\n" +
"function f(x) { return x ? x : new Object(); }");
}
public void testBang3() throws Exception {
testTypes("/** @param {Object} x\n@return {!Object} */\n" +
"function f(x) { return /** @type {!Object} */ (x); }");
}
public void testBang4() throws Exception {
testTypes("/**@param {Object} x\n@param {Object} y\n@return {boolean}*/\n" +
"function f(x, y) {\n" +
"if (typeof x != 'undefined') { return x == y; }\n" +
"else { return x != y; }\n}");
}
public void testBang5() throws Exception {
testTypes("/**@param {Object} x\n@param {Object} y\n@return {boolean}*/\n" +
"function f(x, y) { return !!x && x == y; }");
}
public void testBang6() throws Exception {
testTypes("/** @param {Object?} x\n@return {Object} */\n" +
"function f(x) { return x; }");
}
public void testBang7() throws Exception {
testTypes("/**@param {(Object,string,null)} x\n" +
"@return {(Object,string)}*/function f(x) { return x; }");
}
public void testDefinePropertyOnNullableObject1() throws Exception {
testTypes("/** @type {Object} */ var n = {};\n" +
"/** @type {number} */ n.x = 1;\n" +
"/** @return {boolean} */function f() { return n.x; }",
"inconsistent return type\n" +
"found : number\n" +
"required: boolean");
}
public void testDefinePropertyOnNullableObject2() throws Exception {
testTypes("/** @constructor */ var T = function() {};\n" +
"/** @param {T} t\n@return {boolean} */function f(t) {\n" +
"t.x = 1; return t.x; }",
"inconsistent return type\n" +
"found : number\n" +
"required: boolean");
}
public void testUnknownConstructorInstanceType1() throws Exception {
testTypes("/** @return {Array} */ function g(f) { return new f(); }");
}
public void testUnknownConstructorInstanceType2() throws Exception {
testTypes("function g(f) { return /** @type Array */(new f()); }");
}
public void testUnknownConstructorInstanceType3() throws Exception {
testTypes("function g(f) { var x = new f(); x.a = 1; return x; }");
}
public void testUnknownPrototypeChain() throws Exception {
testTypes("/**\n" +
"* @param {Object} co\n" +
" * @return {Object}\n" +
" */\n" +
"function inst(co) {\n" +
" /** @constructor */\n" +
" var c = function() {};\n" +
" c.prototype = co.prototype;\n" +
" return new c;\n" +
"}");
}
public void testNamespacedConstructor() throws Exception {
Node root = parseAndTypeCheck(
"var goog = {};" +
"/** @constructor */ goog.MyClass = function() {};" +
"/** @return {!goog.MyClass} */ " +
"function foo() { return new goog.MyClass(); }");
JSType typeOfFoo = root.getLastChild().getJSType();
assert(typeOfFoo instanceof FunctionType);
JSType retType = ((FunctionType) typeOfFoo).getReturnType();
assert(retType instanceof ObjectType);
assertEquals("goog.MyClass", ((ObjectType) retType).getReferenceName());
}
public void testComplexNamespace() throws Exception {
String js =
"var goog = {};" +
"goog.foo = {};" +
"goog.foo.bar = 5;";
TypeCheckResult p = parseAndTypeCheckWithScope(js);
// goog type in the scope
JSType googScopeType = p.scope.getVar("goog").getType();
assertTrue(googScopeType instanceof ObjectType);
assertTrue("foo property not present on goog type",
((ObjectType) googScopeType).hasProperty("foo"));
assertFalse("bar property present on goog type",
((ObjectType) googScopeType).hasProperty("bar"));
// goog type on the VAR node
Node varNode = p.root.getFirstChild();
assertEquals(Token.VAR, varNode.getType());
JSType googNodeType = varNode.getFirstChild().getJSType();
assertTrue(googNodeType instanceof ObjectType);
// goog scope type and goog type on VAR node must be the same
assertTrue(googScopeType == googNodeType);
// goog type on the left of the GETPROP node (under fist ASSIGN)
Node getpropFoo1 = varNode.getNext().getFirstChild().getFirstChild();
assertEquals(Token.GETPROP, getpropFoo1.getType());
assertEquals("goog", getpropFoo1.getFirstChild().getString());
JSType googGetpropFoo1Type = getpropFoo1.getFirstChild().getJSType();
assertTrue(googGetpropFoo1Type instanceof ObjectType);
// still the same type as the one on the variable
assertTrue(googGetpropFoo1Type == googScopeType);
// the foo property should be defined on goog
JSType googFooType = ((ObjectType) googScopeType).getPropertyType("foo");
assertTrue(googFooType instanceof ObjectType);
// goog type on the left of the GETPROP lower level node
// (under second ASSIGN)
Node getpropFoo2 = varNode.getNext().getNext()
.getFirstChild().getFirstChild().getFirstChild();
assertEquals(Token.GETPROP, getpropFoo2.getType());
assertEquals("goog", getpropFoo2.getFirstChild().getString());
JSType googGetpropFoo2Type = getpropFoo2.getFirstChild().getJSType();
assertTrue(googGetpropFoo2Type instanceof ObjectType);
// still the same type as the one on the variable
assertTrue(googGetpropFoo2Type == googScopeType);
// goog.foo type on the left of the top-level GETPROP node
// (under second ASSIGN)
JSType googFooGetprop2Type = getpropFoo2.getJSType();
assertTrue("goog.foo incorrectly annotated in goog.foo.bar selection",
googFooGetprop2Type instanceof ObjectType);
ObjectType googFooGetprop2ObjectType = (ObjectType) googFooGetprop2Type;
assertFalse("foo property present on goog.foo type",
googFooGetprop2ObjectType.hasProperty("foo"));
assertTrue("bar property not present on goog.foo type",
googFooGetprop2ObjectType.hasProperty("bar"));
assertTypeEquals("bar property on goog.foo type incorrectly inferred",
NUMBER_TYPE, googFooGetprop2ObjectType.getPropertyType("bar"));
}
public void testAddingMethodsUsingPrototypeIdiomSimpleNamespace()
throws Exception {
Node js1Node = parseAndTypeCheck(
"/** @constructor */function A() {}" +
"A.prototype.m1 = 5");
ObjectType instanceType = getInstanceType(js1Node);
assertEquals(NATIVE_PROPERTIES_COUNT + 1,
instanceType.getPropertiesCount());
checkObjectType(instanceType, "m1", NUMBER_TYPE);
}
public void testAddingMethodsUsingPrototypeIdiomComplexNamespace1()
throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope(
"var goog = {};" +
"goog.A = /** @constructor */function() {};" +
"/** @type number */goog.A.prototype.m1 = 5");
testAddingMethodsUsingPrototypeIdiomComplexNamespace(p);
}
public void testAddingMethodsUsingPrototypeIdiomComplexNamespace2()
throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope(
"var goog = {};" +
"/** @constructor */goog.A = function() {};" +
"/** @type number */goog.A.prototype.m1 = 5");
testAddingMethodsUsingPrototypeIdiomComplexNamespace(p);
}
private void testAddingMethodsUsingPrototypeIdiomComplexNamespace(
TypeCheckResult p) {
ObjectType goog = (ObjectType) p.scope.getVar("goog").getType();
assertEquals(NATIVE_PROPERTIES_COUNT + 1, goog.getPropertiesCount());
JSType googA = goog.getPropertyType("A");
assertNotNull(googA);
assertTrue(googA instanceof FunctionType);
FunctionType googAFunction = (FunctionType) googA;
ObjectType classA = googAFunction.getInstanceType();
assertEquals(NATIVE_PROPERTIES_COUNT + 1, classA.getPropertiesCount());
checkObjectType(classA, "m1", NUMBER_TYPE);
}
public void testAddingMethodsPrototypeIdiomAndObjectLiteralSimpleNamespace()
throws Exception {
Node js1Node = parseAndTypeCheck(
"/** @constructor */function A() {}" +
"A.prototype = {m1: 5, m2: true}");
ObjectType instanceType = getInstanceType(js1Node);
assertEquals(NATIVE_PROPERTIES_COUNT + 2,
instanceType.getPropertiesCount());
checkObjectType(instanceType, "m1", NUMBER_TYPE);
checkObjectType(instanceType, "m2", BOOLEAN_TYPE);
}
public void testDontAddMethodsIfNoConstructor()
throws Exception {
Node js1Node = parseAndTypeCheck(
"function A() {}" +
"A.prototype = {m1: 5, m2: true}");
JSType functionAType = js1Node.getFirstChild().getJSType();
assertEquals("function (): undefined", functionAType.toString());
assertTypeEquals(UNKNOWN_TYPE,
U2U_FUNCTION_TYPE.getPropertyType("m1"));
assertTypeEquals(UNKNOWN_TYPE,
U2U_FUNCTION_TYPE.getPropertyType("m2"));
}
public void testFunctionAssignement() throws Exception {
testTypes("/**" +
"* @param {string} ph0" +
"* @param {string} ph1" +
"* @return {string}" +
"*/" +
"function MSG_CALENDAR_ACCESS_ERROR(ph0, ph1) {return ''}" +
"/** @type {Function} */" +
"var MSG_CALENDAR_ADD_ERROR = MSG_CALENDAR_ACCESS_ERROR;");
}
public void testAddMethodsPrototypeTwoWays() throws Exception {
Node js1Node = parseAndTypeCheck(
"/** @constructor */function A() {}" +
"A.prototype = {m1: 5, m2: true};" +
"A.prototype.m3 = 'third property!';");
ObjectType instanceType = getInstanceType(js1Node);
assertEquals("A", instanceType.toString());
assertEquals(NATIVE_PROPERTIES_COUNT + 3,
instanceType.getPropertiesCount());
checkObjectType(instanceType, "m1", NUMBER_TYPE);
checkObjectType(instanceType, "m2", BOOLEAN_TYPE);
checkObjectType(instanceType, "m3", STRING_TYPE);
}
public void testPrototypePropertyTypes() throws Exception {
Node js1Node = parseAndTypeCheck(
"/** @constructor */function A() {\n" +
" /** @type string */ this.m1;\n" +
" /** @type Object? */ this.m2 = {};\n" +
" /** @type boolean */ this.m3;\n" +
"}\n" +
"/** @type string */ A.prototype.m4;\n" +
"/** @type number */ A.prototype.m5 = 0;\n" +
"/** @type boolean */ A.prototype.m6;\n");
ObjectType instanceType = getInstanceType(js1Node);
assertEquals(NATIVE_PROPERTIES_COUNT + 6,
instanceType.getPropertiesCount());
checkObjectType(instanceType, "m1", STRING_TYPE);
checkObjectType(instanceType, "m2",
createUnionType(OBJECT_TYPE, NULL_TYPE));
checkObjectType(instanceType, "m3", BOOLEAN_TYPE);
checkObjectType(instanceType, "m4", STRING_TYPE);
checkObjectType(instanceType, "m5", NUMBER_TYPE);
checkObjectType(instanceType, "m6", BOOLEAN_TYPE);
}
public void testValueTypeBuiltInPrototypePropertyType() throws Exception {
Node node = parseAndTypeCheck("\"x\".charAt(0)");
assertTypeEquals(STRING_TYPE, node.getFirstChild().getFirstChild().getJSType());
}
public void testDeclareBuiltInConstructor() throws Exception {
// Built-in prototype properties should be accessible
// even if the built-in constructor is declared.
Node node = parseAndTypeCheck(
"/** @constructor */ var String = function(opt_str) {};\n" +
"(new String(\"x\")).charAt(0)");
assertTypeEquals(STRING_TYPE, node.getLastChild().getFirstChild().getJSType());
}
public void testExtendBuiltInType1() throws Exception {
String externs =
"/** @constructor */ var String = function(opt_str) {};\n" +
"/**\n" +
"* @param {number} start\n" +
"* @param {number} opt_length\n" +
"* @return {string}\n" +
"*/\n" +
"String.prototype.substr = function(start, opt_length) {};\n";
Node n1 = parseAndTypeCheck(externs + "(new String(\"x\")).substr(0,1);");
assertTypeEquals(STRING_TYPE, n1.getLastChild().getFirstChild().getJSType());
}
public void testExtendBuiltInType2() throws Exception {
String externs =
"/** @constructor */ var String = function(opt_str) {};\n" +
"/**\n" +
"* @param {number} start\n" +
"* @param {number} opt_length\n" +
"* @return {string}\n" +
"*/\n" +
"String.prototype.substr = function(start, opt_length) {};\n";
Node n2 = parseAndTypeCheck(externs + "\"x\".substr(0,1);");
assertTypeEquals(STRING_TYPE, n2.getLastChild().getFirstChild().getJSType());
}
public void testExtendFunction1() throws Exception {
Node n = parseAndTypeCheck("/**@return {number}*/Function.prototype.f = " +
"function() { return 1; };\n" +
"(new Function()).f();");
JSType type = n.getLastChild().getLastChild().getJSType();
assertTypeEquals(NUMBER_TYPE, type);
}
public void testExtendFunction2() throws Exception {
Node n = parseAndTypeCheck("/**@return {number}*/Function.prototype.f = " +
"function() { return 1; };\n" +
"(function() {}).f();");
JSType type = n.getLastChild().getLastChild().getJSType();
assertTypeEquals(NUMBER_TYPE, type);
}
public void testInheritanceCheck1() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"Sub.prototype.foo = function() {};");
}
public void testInheritanceCheck2() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override */Sub.prototype.foo = function() {};",
"property foo not defined on any superclass of Sub");
}
public void testInheritanceCheck3() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"Super.prototype.foo = function() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"Sub.prototype.foo = function() {};",
"property foo already defined on superclass Super; " +
"use @override to override it");
}
public void testInheritanceCheck4() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"Super.prototype.foo = function() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override */Sub.prototype.foo = function() {};");
}
public void testInheritanceCheck5() throws Exception {
testTypes(
"/** @constructor */function Root() {};" +
"Root.prototype.foo = function() {};" +
"/** @constructor\n @extends {Root} */function Super() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"Sub.prototype.foo = function() {};",
"property foo already defined on superclass Root; " +
"use @override to override it");
}
public void testInheritanceCheck6() throws Exception {
testTypes(
"/** @constructor */function Root() {};" +
"Root.prototype.foo = function() {};" +
"/** @constructor\n @extends {Root} */function Super() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override */Sub.prototype.foo = function() {};");
}
public void testInheritanceCheck7() throws Exception {
testTypes(
"var goog = {};" +
"/** @constructor */goog.Super = function() {};" +
"goog.Super.prototype.foo = 3;" +
"/** @constructor\n @extends {goog.Super} */goog.Sub = function() {};" +
"goog.Sub.prototype.foo = 5;");
}
public void testInheritanceCheck8() throws Exception {
testTypes(
"var goog = {};" +
"/** @constructor */goog.Super = function() {};" +
"goog.Super.prototype.foo = 3;" +
"/** @constructor\n @extends {goog.Super} */goog.Sub = function() {};" +
"/** @override */goog.Sub.prototype.foo = 5;");
}
public void testInheritanceCheck9_1() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"Super.prototype.foo = function() { return 3; };" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override\n @return {number} */Sub.prototype.foo =\n" +
"function() { return 1; };");
}
public void testInheritanceCheck9_2() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"/** @return {number} */" +
"Super.prototype.foo = function() { return 1; };" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override */Sub.prototype.foo =\n" +
"function() {};");
}
public void testInheritanceCheck9_3() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"/** @return {number} */" +
"Super.prototype.foo = function() { return 1; };" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override\n @return {string} */Sub.prototype.foo =\n" +
"function() { return \"some string\" };",
"mismatch of the foo property type and the type of the property it " +
"overrides from superclass Super\n" +
"original: function (this:Super): number\n" +
"override: function (this:Sub): string");
}
public void testInheritanceCheck10_1() throws Exception {
testTypes(
"/** @constructor */function Root() {};" +
"Root.prototype.foo = function() { return 3; };" +
"/** @constructor\n @extends {Root} */function Super() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override\n @return {number} */Sub.prototype.foo =\n" +
"function() { return 1; };");
}
public void testInheritanceCheck10_2() throws Exception {
testTypes(
"/** @constructor */function Root() {};" +
"/** @return {number} */" +
"Root.prototype.foo = function() { return 1; };" +
"/** @constructor\n @extends {Root} */function Super() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override */Sub.prototype.foo =\n" +
"function() {};");
}
public void testInheritanceCheck10_3() throws Exception {
testTypes(
"/** @constructor */function Root() {};" +
"/** @return {number} */" +
"Root.prototype.foo = function() { return 1; };" +
"/** @constructor\n @extends {Root} */function Super() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override\n @return {string} */Sub.prototype.foo =\n" +
"function() { return \"some string\" };",
"mismatch of the foo property type and the type of the property it " +
"overrides from superclass Root\n" +
"original: function (this:Root): number\n" +
"override: function (this:Sub): string");
}
public void testInterfaceInheritanceCheck11() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"/** @param {number} bar */Super.prototype.foo = function(bar) {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override\n @param {string} bar */Sub.prototype.foo =\n" +
"function(bar) {};",
"mismatch of the foo property type and the type of the property it " +
"overrides from superclass Super\n" +
"original: function (this:Super, number): undefined\n" +
"override: function (this:Sub, string): undefined");
}
public void testInheritanceCheck12() throws Exception {
testTypes(
"var goog = {};" +
"/** @constructor */goog.Super = function() {};" +
"goog.Super.prototype.foo = 3;" +
"/** @constructor\n @extends {goog.Super} */goog.Sub = function() {};" +
"/** @override */goog.Sub.prototype.foo = \"some string\";");
}
public void testInheritanceCheck13() throws Exception {
testTypes(
"var goog = {};\n" +
"/** @constructor\n @extends {goog.Missing} */function Sub() {};" +
"/** @override */Sub.prototype.foo = function() {};",
"Bad type annotation. Unknown type goog.Missing");
}
public void testInheritanceCheck14() throws Exception {
testClosureTypes(
"var goog = {};\n" +
"/** @constructor\n @extends {goog.Missing} */\n" +
"goog.Super = function() {};\n" +
"/** @constructor\n @extends {goog.Super} */function Sub() {};" +
"/** @override */Sub.prototype.foo = function() {};",
"Bad type annotation. Unknown type goog.Missing");
}
public void testInheritanceCheck15() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"/** @param {number} bar */Super.prototype.foo;" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override\n @param {number} bar */Sub.prototype.foo =\n" +
"function(bar) {};");
}
public void testInheritanceCheck16() throws Exception {
testTypes(
"var goog = {};" +
"/** @constructor */goog.Super = function() {};" +
"/** @type {number} */ goog.Super.prototype.foo = 3;" +
"/** @constructor\n @extends {goog.Super} */goog.Sub = function() {};" +
"/** @type {number} */ goog.Sub.prototype.foo = 5;",
"property foo already defined on superclass goog.Super; " +
"use @override to override it");
}
public void testInheritanceCheck17() throws Exception {
// Make sure this warning still works, even when there's no
// @override tag.
reportMissingOverrides = CheckLevel.OFF;
testTypes(
"var goog = {};" +
"/** @constructor */goog.Super = function() {};" +
"/** @param {number} x */ goog.Super.prototype.foo = function(x) {};" +
"/** @constructor\n @extends {goog.Super} */goog.Sub = function() {};" +
"/** @param {string} x */ goog.Sub.prototype.foo = function(x) {};",
"mismatch of the foo property type and the type of the property it " +
"overrides from superclass goog.Super\n" +
"original: function (this:goog.Super, number): undefined\n" +
"override: function (this:goog.Sub, string): undefined");
}
public void testInterfacePropertyOverride1() throws Exception {
testTypes(
"/** @interface */function Super() {};" +
"/** @desc description */Super.prototype.foo = function() {};" +
"/** @interface\n @extends {Super} */function Sub() {};" +
"/** @desc description */Sub.prototype.foo = function() {};");
}
public void testInterfacePropertyOverride2() throws Exception {
testTypes(
"/** @interface */function Root() {};" +
"/** @desc description */Root.prototype.foo = function() {};" +
"/** @interface\n @extends {Root} */function Super() {};" +
"/** @interface\n @extends {Super} */function Sub() {};" +
"/** @desc description */Sub.prototype.foo = function() {};");
}
public void testInterfaceInheritanceCheck1() throws Exception {
testTypes(
"/** @interface */function Super() {};" +
"/** @desc description */Super.prototype.foo = function() {};" +
"/** @constructor\n @implements {Super} */function Sub() {};" +
"Sub.prototype.foo = function() {};",
"property foo already defined on interface Super; use @override to " +
"override it");
}
public void testInterfaceInheritanceCheck2() throws Exception {
testTypes(
"/** @interface */function Super() {};" +
"/** @desc description */Super.prototype.foo = function() {};" +
"/** @constructor\n @implements {Super} */function Sub() {};" +
"/** @override */Sub.prototype.foo = function() {};");
}
public void testInterfaceInheritanceCheck3() throws Exception {
testTypes(
"/** @interface */function Root() {};" +
"/** @return {number} */Root.prototype.foo = function() {};" +
"/** @interface\n @extends {Root} */function Super() {};" +
"/** @constructor\n @implements {Super} */function Sub() {};" +
"/** @return {number} */Sub.prototype.foo = function() { return 1;};",
"property foo already defined on interface Root; use @override to " +
"override it");
}
public void testInterfaceInheritanceCheck4() throws Exception {
testTypes(
"/** @interface */function Root() {};" +
"/** @return {number} */Root.prototype.foo = function() {};" +
"/** @interface\n @extends {Root} */function Super() {};" +
"/** @constructor\n @implements {Super} */function Sub() {};" +
"/** @override\n * @return {number} */Sub.prototype.foo =\n" +
"function() { return 1;};");
}
public void testInterfaceInheritanceCheck5() throws Exception {
testTypes(
"/** @interface */function Super() {};" +
"/** @return {string} */Super.prototype.foo = function() {};" +
"/** @constructor\n @implements {Super} */function Sub() {};" +
"/** @override\n @return {number} */Sub.prototype.foo =\n" +
"function() { return 1; };",
"mismatch of the foo property type and the type of the property it " +
"overrides from interface Super\n" +
"original: function (this:Super): string\n" +
"override: function (this:Sub): number");
}
public void testInterfaceInheritanceCheck6() throws Exception {
testTypes(
"/** @interface */function Root() {};" +
"/** @return {string} */Root.prototype.foo = function() {};" +
"/** @interface\n @extends {Root} */function Super() {};" +
"/** @constructor\n @implements {Super} */function Sub() {};" +
"/** @override\n @return {number} */Sub.prototype.foo =\n" +
"function() { return 1; };",
"mismatch of the foo property type and the type of the property it " +
"overrides from interface Root\n" +
"original: function (this:Root): string\n" +
"override: function (this:Sub): number");
}
public void testInterfaceInheritanceCheck7() throws Exception {
testTypes(
"/** @interface */function Super() {};" +
"/** @param {number} bar */Super.prototype.foo = function(bar) {};" +
"/** @constructor\n @implements {Super} */function Sub() {};" +
"/** @override\n @param {string} bar */Sub.prototype.foo =\n" +
"function(bar) {};",
"mismatch of the foo property type and the type of the property it " +
"overrides from interface Super\n" +
"original: function (this:Super, number): undefined\n" +
"override: function (this:Sub, string): undefined");
}
public void testInterfaceInheritanceCheck8() throws Exception {
testTypes(
"/** @constructor\n @implements {Super} */function Sub() {};" +
"/** @override */Sub.prototype.foo = function() {};",
new String[] {
"Bad type annotation. Unknown type Super",
"property foo not defined on any superclass of Sub"
});
}
public void testInterfaceInheritanceCheck9() throws Exception {
testTypes(
"/** @interface */ function I() {}" +
"/** @return {number} */ I.prototype.bar = function() {};" +
"/** @constructor */ function F() {}" +
"/** @return {number} */ F.prototype.bar = function() {return 3; };" +
"/** @return {number} */ F.prototype.foo = function() {return 3; };" +
"/** @constructor \n * @extends {F} \n * @implements {I} */ " +
"function G() {}" +
"/** @return {string} */ function f() { return new G().bar(); }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testInterfaceInheritanceCheck10() throws Exception {
testTypes(
"/** @interface */ function I() {}" +
"/** @return {number} */ I.prototype.bar = function() {};" +
"/** @constructor */ function F() {}" +
"/** @return {number} */ F.prototype.foo = function() {return 3; };" +
"/** @constructor \n * @extends {F} \n * @implements {I} */ " +
"function G() {}" +
"/** @return {number} \n * @override */ " +
"G.prototype.bar = G.prototype.foo;" +
"/** @return {string} */ function f() { return new G().bar(); }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testInterfaceInheritanceCheck12() throws Exception {
testTypes(
"/** @interface */ function I() {};\n" +
"/** @type {string} */ I.prototype.foobar;\n" +
"/** \n * @constructor \n * @implements {I} */\n" +
"function C() {\n" +
"/** \n * @type {number} */ this.foobar = 2;};\n" +
"/** @type {I} */ \n var test = new C(); alert(test.foobar);",
"mismatch of the foobar property type and the type of the property" +
" it overrides from interface I\n" +
"original: string\n" +
"override: number");
}
public void testInterfaceInheritanceCheck13() throws Exception {
testTypes(
"function abstractMethod() {};\n" +
"/** @interface */var base = function() {};\n" +
"/** @extends {base} \n @interface */ var Int = function() {}\n" +
"/** @type {{bar : !Function}} */ var x; \n" +
"/** @type {!Function} */ base.prototype.bar = abstractMethod; \n" +
"/** @type {Int} */ var foo;\n" +
"foo.bar();");
}
public void testInterfacePropertyNotImplemented() throws Exception {
testTypes(
"/** @interface */function Int() {};" +
"/** @desc description */Int.prototype.foo = function() {};" +
"/** @constructor\n @implements {Int} */function Foo() {};",
"property foo on interface Int is not implemented by type Foo");
}
public void testInterfacePropertyNotImplemented2() throws Exception {
testTypes(
"/** @interface */function Int() {};" +
"/** @desc description */Int.prototype.foo = function() {};" +
"/** @interface \n @extends {Int} */function Int2() {};" +
"/** @constructor\n @implements {Int2} */function Foo() {};",
"property foo on interface Int is not implemented by type Foo");
}
public void testStubConstructorImplementingInterface() throws Exception {
// This does not throw a warning for unimplemented property because Foo is
// just a stub.
testTypes(
// externs
"/** @interface */ function Int() {}\n" +
"/** @desc description */Int.prototype.foo = function() {};" +
"/** @constructor \n @implements {Int} */ var Foo;\n",
"", null, false);
}
public void testObjectLiteral() throws Exception {
Node n = parseAndTypeCheck("var a = {m1: 7, m2: 'hello'}");
Node nameNode = n.getFirstChild().getFirstChild();
Node objectNode = nameNode.getFirstChild();
// node extraction
assertEquals(Token.NAME, nameNode.getType());
assertEquals(Token.OBJECTLIT, objectNode.getType());
// value's type
ObjectType objectType =
(ObjectType) objectNode.getJSType();
assertTypeEquals(NUMBER_TYPE, objectType.getPropertyType("m1"));
assertTypeEquals(STRING_TYPE, objectType.getPropertyType("m2"));
// variable's type
assertTypeEquals(objectType, nameNode.getJSType());
}
public void testObjectLiteralDeclaration1() throws Exception {
testTypes(
"var x = {" +
"/** @type {boolean} */ abc: true," +
"/** @type {number} */ 'def': 0," +
"/** @type {string} */ 3: 'fgh'" +
"};");
}
public void testObjectLiteralDeclaration2() throws Exception {
testTypes(
"var x = {" +
" /** @type {boolean} */ abc: true" +
"};" +
"x.abc = 0;",
"assignment to property abc of x\n" +
"found : number\n" +
"required: boolean");
}
public void testObjectLiteralDeclaration3() throws Exception {
testTypes(
"/** @param {{foo: !Function}} x */ function f(x) {}" +
"f({foo: function() {}});");
}
public void testObjectLiteralDeclaration4() throws Exception {
testClosureTypes(
"var x = {" +
" /** @param {boolean} x */ abc: function(x) {}" +
"};" +
"/**\n" +
" * @param {string} x\n" +
" * @suppress {duplicate}\n" +
" */ x.abc = function(x) {};",
"assignment to property abc of x\n" +
"found : function (string): undefined\n" +
"required: function (boolean): undefined");
// TODO(user): suppress {duplicate} currently also silence the
// redefining type error in the TypeValidator. Maybe it needs
// a new suppress name instead?
}
public void testObjectLiteralDeclaration5() throws Exception {
testTypes(
"var x = {" +
" /** @param {boolean} x */ abc: function(x) {}" +
"};" +
"/**\n" +
" * @param {boolean} x\n" +
" * @suppress {duplicate}\n" +
" */ x.abc = function(x) {};");
}
public void testObjectLiteralDeclaration6() throws Exception {
testTypes(
"var x = {};" +
"/**\n" +
" * @param {boolean} x\n" +
" * @suppress {duplicate}\n" +
" */ x.abc = function(x) {};" +
"x = {" +
" /**\n" +
" * @param {boolean} x\n" +
" * @suppress {duplicate}\n" +
" */" +
" abc: function(x) {}" +
"};");
}
public void testObjectLiteralDeclaration7() throws Exception {
testTypes(
"var x = {};" +
"/**\n" +
" * @type {function(boolean): undefined}\n" +
" */ x.abc = function(x) {};" +
"x = {" +
" /**\n" +
" * @param {boolean} x\n" +
" * @suppress {duplicate}\n" +
" */" +
" abc: function(x) {}" +
"};");
}
public void testCallDateConstructorAsFunction() throws Exception {
// ECMA-262 15.9.2: When Date is called as a function rather than as a
// constructor, it returns a string.
Node n = parseAndTypeCheck("Date()");
assertTypeEquals(STRING_TYPE, n.getFirstChild().getFirstChild().getJSType());
}
// According to ECMA-262, Error & Array function calls are equivalent to
// constructor calls.
public void testCallErrorConstructorAsFunction() throws Exception {
Node n = parseAndTypeCheck("Error('x')");
assertTypeEquals(ERROR_TYPE,
n.getFirstChild().getFirstChild().getJSType());
}
public void testCallArrayConstructorAsFunction() throws Exception {
Node n = parseAndTypeCheck("Array()");
assertTypeEquals(ARRAY_TYPE,
n.getFirstChild().getFirstChild().getJSType());
}
public void testPropertyTypeOfUnionType() throws Exception {
testTypes("var a = {};" +
"/** @constructor */ a.N = function() {};\n" +
"a.N.prototype.p = 1;\n" +
"/** @constructor */ a.S = function() {};\n" +
"a.S.prototype.p = 'a';\n" +
"/** @param {!a.N|!a.S} x\n@return {string} */\n" +
"var f = function(x) { return x.p; };",
"inconsistent return type\n" +
"found : (number|string)\n" +
"required: string");
}
// TODO(user): We should flag these as invalid. This will probably happen
// when we make sure the interface is never referenced outside of its
// definition. We might want more specific and helpful error messages.
//public void testWarningOnInterfacePrototype() throws Exception {
// testTypes("/** @interface */ u.T = function() {};\n" +
// "/** @return {number} */ u.T.prototype = function() { };",
// "e of its definition");
//}
//
//public void testBadPropertyOnInterface1() throws Exception {
// testTypes("/** @interface */ u.T = function() {};\n" +
// "/** @return {number} */ u.T.f = function() { return 1;};",
// "cannot reference an interface outside of its definition");
//}
//
//public void testBadPropertyOnInterface2() throws Exception {
// testTypes("/** @interface */ function T() {};\n" +
// "/** @return {number} */ T.f = function() { return 1;};",
// "cannot reference an interface outside of its definition");
//}
//
//public void testBadPropertyOnInterface3() throws Exception {
// testTypes("/** @interface */ u.T = function() {}; u.T.x",
// "cannot reference an interface outside of its definition");
//}
//
//public void testBadPropertyOnInterface4() throws Exception {
// testTypes("/** @interface */ function T() {}; T.x;",
// "cannot reference an interface outside of its definition");
//}
public void testAnnotatedPropertyOnInterface1() throws Exception {
// For interfaces we must allow function definitions that don't have a
// return statement, even though they declare a returned type.
testTypes("/** @interface */ u.T = function() {};\n" +
"/** @return {number} */ u.T.prototype.f = function() {};");
}
public void testAnnotatedPropertyOnInterface2() throws Exception {
testTypes("/** @interface */ u.T = function() {};\n" +
"/** @return {number} */ u.T.prototype.f = function() { };");
}
public void testAnnotatedPropertyOnInterface3() throws Exception {
testTypes("/** @interface */ function T() {};\n" +
"/** @return {number} */ T.prototype.f = function() { };");
}
public void testAnnotatedPropertyOnInterface4() throws Exception {
testTypes(
CLOSURE_DEFS +
"/** @interface */ function T() {};\n" +
"/** @return {number} */ T.prototype.f = goog.abstractMethod;");
}
// TODO(user): If we want to support this syntax we have to warn about
// missing annotations.
//public void testWarnUnannotatedPropertyOnInterface1() throws Exception {
// testTypes("/** @interface */ u.T = function () {}; u.T.prototype.x;",
// "interface property x is not annotated");
//}
//
//public void testWarnUnannotatedPropertyOnInterface2() throws Exception {
// testTypes("/** @interface */ function T() {}; T.prototype.x;",
// "interface property x is not annotated");
//}
public void testWarnUnannotatedPropertyOnInterface5() throws Exception {
testTypes("/** @interface */ u.T = function () {};\n" +
"/** @desc x does something */u.T.prototype.x = function() {};");
}
public void testWarnUnannotatedPropertyOnInterface6() throws Exception {
testTypes("/** @interface */ function T() {};\n" +
"/** @desc x does something */T.prototype.x = function() {};");
}
// TODO(user): If we want to support this syntax we have to warn about
// the invalid type of the interface member.
//public void testWarnDataPropertyOnInterface1() throws Exception {
// testTypes("/** @interface */ u.T = function () {};\n" +
// "/** @type {number} */u.T.prototype.x;",
// "interface members can only be plain functions");
//}
public void testDataPropertyOnInterface1() throws Exception {
testTypes("/** @interface */ function T() {};\n" +
"/** @type {number} */T.prototype.x;");
}
public void testDataPropertyOnInterface2() throws Exception {
reportMissingOverrides = CheckLevel.OFF;
testTypes("/** @interface */ function T() {};\n" +
"/** @type {number} */T.prototype.x;\n" +
"/** @constructor \n" +
" * @implements {T} \n" +
" */\n" +
"function C() {}\n" +
"C.prototype.x = 'foo';",
"mismatch of the x property type and the type of the property it " +
"overrides from interface T\n" +
"original: number\n" +
"override: string");
}
public void testDataPropertyOnInterface3() throws Exception {
testTypes("/** @interface */ function T() {};\n" +
"/** @type {number} */T.prototype.x;\n" +
"/** @constructor \n" +
" * @implements {T} \n" +
" */\n" +
"function C() {}\n" +
"/** @override */\n" +
"C.prototype.x = 'foo';",
"mismatch of the x property type and the type of the property it " +
"overrides from interface T\n" +
"original: number\n" +
"override: string");
}
public void testDataPropertyOnInterface4() throws Exception {
testTypes("/** @interface */ function T() {};\n" +
"/** @type {number} */T.prototype.x;\n" +
"/** @constructor \n" +
" * @implements {T} \n" +
" */\n" +
"function C() { /** @type {string} */ \n this.x = 'foo'; }\n",
"mismatch of the x property type and the type of the property it " +
"overrides from interface T\n" +
"original: number\n" +
"override: string");
}
public void testWarnDataPropertyOnInterface3() throws Exception {
testTypes("/** @interface */ u.T = function () {};\n" +
"/** @type {number} */u.T.prototype.x = 1;",
"interface members can only be empty property declarations, "
+ "empty functions, or goog.abstractMethod");
}
public void testWarnDataPropertyOnInterface4() throws Exception {
testTypes("/** @interface */ function T() {};\n" +
"/** @type {number} */T.prototype.x = 1;",
"interface members can only be empty property declarations, "
+ "empty functions, or goog.abstractMethod");
}
// TODO(user): If we want to support this syntax we should warn about the
// mismatching types in the two tests below.
//public void testErrorMismatchingPropertyOnInterface1() throws Exception {
// testTypes("/** @interface */ u.T = function () {};\n" +
// "/** @param {Number} foo */u.T.prototype.x =\n" +
// "/** @param {String} foo */function(foo) {};",
// "found : \n" +
// "required: ");
//}
//
//public void testErrorMismatchingPropertyOnInterface2() throws Exception {
// testTypes("/** @interface */ function T() {};\n" +
// "/** @return {number} */T.prototype.x =\n" +
// "/** @return {string} */function() {};",
// "found : \n" +
// "required: ");
//}
// TODO(user): We should warn about this (bar is missing an annotation). We
// probably don't want to warn about all missing parameter annotations, but
// we should be as strict as possible regarding interfaces.
//public void testErrorMismatchingPropertyOnInterface3() throws Exception {
// testTypes("/** @interface */ u.T = function () {};\n" +
// "/** @param {Number} foo */u.T.prototype.x =\n" +
// "function(foo, bar) {};",
// "found : \n" +
// "required: ");
//}
public void testErrorMismatchingPropertyOnInterface4() throws Exception {
testTypes("/** @interface */ u.T = function () {};\n" +
"/** @param {Number} foo */u.T.prototype.x =\n" +
"function() {};",
"parameter foo does not appear in u.T.prototype.x's parameter list");
}
public void testErrorMismatchingPropertyOnInterface5() throws Exception {
testTypes("/** @interface */ function T() {};\n" +
"/** @type {number} */T.prototype.x = function() { };",
"assignment to property x of T.prototype\n" +
"found : function (): undefined\n" +
"required: number");
}
public void testErrorMismatchingPropertyOnInterface6() throws Exception {
testClosureTypesMultipleWarnings(
"/** @interface */ function T() {};\n" +
"/** @return {number} */T.prototype.x = 1",
Lists.newArrayList(
"assignment to property x of T.prototype\n" +
"found : number\n" +
"required: function (this:T): number",
"interface members can only be empty property declarations, " +
"empty functions, or goog.abstractMethod"));
}
public void testInterfaceNonEmptyFunction() throws Exception {
testTypes("/** @interface */ function T() {};\n" +
"T.prototype.x = function() { return 'foo'; }",
"interface member functions must have an empty body"
);
}
public void testDoubleNestedInterface() throws Exception {
testTypes("/** @interface */ var I1 = function() {};\n" +
"/** @interface */ I1.I2 = function() {};\n" +
"/** @interface */ I1.I2.I3 = function() {};\n");
}
public void testStaticDataPropertyOnNestedInterface() throws Exception {
testTypes("/** @interface */ var I1 = function() {};\n" +
"/** @interface */ I1.I2 = function() {};\n" +
"/** @type {number} */ I1.I2.x = 1;\n");
}
public void testInterfaceInstantiation() throws Exception {
testTypes("/** @interface */var f = function(){}; new f",
"cannot instantiate non-constructor");
}
public void testPrototypeLoop() throws Exception {
testClosureTypesMultipleWarnings(
suppressMissingProperty("foo") +
"/** @constructor \n * @extends {T} */var T = function() {};" +
"alert((new T).foo);",
Lists.newArrayList(
"Parse error. Cycle detected in inheritance chain of type T",
"Could not resolve type in @extends tag of T"));
}
public void testImplementsLoop() throws Exception {
testClosureTypesMultipleWarnings(
suppressMissingProperty("foo") +
"/** @constructor \n * @implements {T} */var T = function() {};" +
"alert((new T).foo);",
Lists.newArrayList(
"Parse error. Cycle detected in inheritance chain of type T"));
}
public void testImplementsExtendsLoop() throws Exception {
testClosureTypesMultipleWarnings(
suppressMissingProperty("foo") +
"/** @constructor \n * @implements {F} */var G = function() {};" +
"/** @constructor \n * @extends {G} */var F = function() {};" +
"alert((new F).foo);",
Lists.newArrayList(
"Parse error. Cycle detected in inheritance chain of type F"));
}
public void testInterfaceExtendsLoop() throws Exception {
// TODO(user): This should give a cycle in inheritance graph error,
// not a cannot resolve error.
testClosureTypesMultipleWarnings(
suppressMissingProperty("foo") +
"/** @interface \n * @extends {F} */var G = function() {};" +
"/** @interface \n * @extends {G} */var F = function() {};",
Lists.newArrayList(
"Could not resolve type in @extends tag of G"));
}
public void testConversionFromInterfaceToRecursiveConstructor()
throws Exception {
testClosureTypesMultipleWarnings(
suppressMissingProperty("foo") +
"/** @interface */ var OtherType = function() {}\n" +
"/** @implements {MyType} \n * @constructor */\n" +
"var MyType = function() {}\n" +
"/** @type {MyType} */\n" +
"var x = /** @type {!OtherType} */ (new Object());",
Lists.newArrayList(
"Parse error. Cycle detected in inheritance chain of type MyType",
"initializing variable\n" +
"found : OtherType\n" +
"required: (MyType|null)"));
}
public void testDirectPrototypeAssign() throws Exception {
// For now, we just ignore @type annotations on the prototype.
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @constructor */ function Bar() {}" +
"/** @type {Array} */ Bar.prototype = new Foo()");
}
// In all testResolutionViaRegistry* tests, since u is unknown, u.T can only
// be resolved via the registry and not via properties.
public void testResolutionViaRegistry1() throws Exception {
testTypes("/** @constructor */ u.T = function() {};\n" +
"/** @type {(number|string)} */ u.T.prototype.a;\n" +
"/**\n" +
"* @param {u.T} t\n" +
"* @return {string}\n" +
"*/\n" +
"var f = function(t) { return t.a; };",
"inconsistent return type\n" +
"found : (number|string)\n" +
"required: string");
}
public void testResolutionViaRegistry2() throws Exception {
testTypes(
"/** @constructor */ u.T = function() {" +
" this.a = 0; };\n" +
"/**\n" +
"* @param {u.T} t\n" +
"* @return {string}\n" +
"*/\n" +
"var f = function(t) { return t.a; };",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testResolutionViaRegistry3() throws Exception {
testTypes("/** @constructor */ u.T = function() {};\n" +
"/** @type {(number|string)} */ u.T.prototype.a = 0;\n" +
"/**\n" +
"* @param {u.T} t\n" +
"* @return {string}\n" +
"*/\n" +
"var f = function(t) { return t.a; };",
"inconsistent return type\n" +
"found : (number|string)\n" +
"required: string");
}
public void testResolutionViaRegistry4() throws Exception {
testTypes("/** @constructor */ u.A = function() {};\n" +
"/**\n* @constructor\n* @extends {u.A}\n*/\nu.A.A = function() {}\n;" +
"/**\n* @constructor\n* @extends {u.A}\n*/\nu.A.B = function() {};\n" +
"var ab = new u.A.B();\n" +
"/** @type {!u.A} */ var a = ab;\n" +
"/** @type {!u.A.A} */ var aa = ab;\n",
"initializing variable\n" +
"found : u.A.B\n" +
"required: u.A.A");
}
public void testResolutionViaRegistry5() throws Exception {
Node n = parseAndTypeCheck("/** @constructor */ u.T = function() {}; u.T");
JSType type = n.getLastChild().getLastChild().getJSType();
assertFalse(type.isUnknownType());
assertTrue(type instanceof FunctionType);
assertEquals("u.T",
((FunctionType) type).getInstanceType().getReferenceName());
}
public void testGatherProperyWithoutAnnotation1() throws Exception {
Node n = parseAndTypeCheck("/** @constructor */ var T = function() {};" +
"/** @type {!T} */var t; t.x; t;");
JSType type = n.getLastChild().getLastChild().getJSType();
assertFalse(type.isUnknownType());
assertTrue(type instanceof ObjectType);
ObjectType objectType = (ObjectType) type;
assertFalse(objectType.hasProperty("x"));
Asserts.assertTypeCollectionEquals(
Lists.newArrayList(objectType),
registry.getTypesWithProperty("x"));
}
public void testGatherProperyWithoutAnnotation2() throws Exception {
TypeCheckResult ns =
parseAndTypeCheckWithScope("/** @type {!Object} */var t; t.x; t;");
Node n = ns.root;
Scope s = ns.scope;
JSType type = n.getLastChild().getLastChild().getJSType();
assertFalse(type.isUnknownType());
assertTypeEquals(type, OBJECT_TYPE);
assertTrue(type instanceof ObjectType);
ObjectType objectType = (ObjectType) type;
assertFalse(objectType.hasProperty("x"));
Asserts.assertTypeCollectionEquals(
Lists.newArrayList(OBJECT_TYPE),
registry.getTypesWithProperty("x"));
}
public void testFunctionMasksVariableBug() throws Exception {
testTypes("var x = 4; var f = function x(b) { return b ? 1 : x(true); };",
"function x masks variable (IE bug)");
}
public void testDfa1() throws Exception {
testTypes("var x = null;\n x = 1;\n /** @type number */ var y = x;");
}
public void testDfa2() throws Exception {
testTypes("function u() {}\n" +
"/** @return {number} */ function f() {\nvar x = 'todo';\n" +
"if (u()) { x = 1; } else { x = 2; } return x;\n}");
}
public void testDfa3() throws Exception {
testTypes("function u() {}\n" +
"/** @return {number} */ function f() {\n" +
"/** @type {number|string} */ var x = 'todo';\n" +
"if (u()) { x = 1; } else { x = 2; } return x;\n}");
}
public void testDfa4() throws Exception {
testTypes("/** @param {Date?} d */ function f(d) {\n" +
"if (!d) { return; }\n" +
"/** @type {!Date} */ var e = d;\n}");
}
public void testDfa5() throws Exception {
testTypes("/** @return {string?} */ function u() {return 'a';}\n" +
"/** @param {string?} x\n@return {string} */ function f(x) {\n" +
"while (!x) { x = u(); }\nreturn x;\n}");
}
public void testDfa6() throws Exception {
testTypes("/** @return {Object?} */ function u() {return {};}\n" +
"/** @param {Object?} x */ function f(x) {\n" +
"while (x) { x = u(); if (!x) { x = u(); } }\n}");
}
public void testDfa7() throws Exception {
testTypes("/** @constructor */ var T = function() {};\n" +
"/** @type {Date?} */ T.prototype.x = null;\n" +
"/** @param {!T} t */ function f(t) {\n" +
"if (!t.x) { return; }\n" +
"/** @type {!Date} */ var e = t.x;\n}");
}
public void testDfa8() throws Exception {
testTypes("/** @constructor */ var T = function() {};\n" +
"/** @type {number|string} */ T.prototype.x = '';\n" +
"function u() {}\n" +
"/** @param {!T} t\n@return {number} */ function f(t) {\n" +
"if (u()) { t.x = 1; } else { t.x = 2; } return t.x;\n}");
}
public void testDfa9() throws Exception {
testTypes("function f() {\n/** @type {string?} */var x;\nx = null;\n" +
"if (x == null) { return 0; } else { return 1; } }",
"condition always evaluates to true\n" +
"left : null\n" +
"right: null");
}
public void testDfa10() throws Exception {
testTypes("/** @param {null} x */ function g(x) {}" +
"/** @param {string?} x */function f(x) {\n" +
"if (!x) { x = ''; }\n" +
"if (g(x)) { return 0; } else { return 1; } }",
"actual parameter 1 of g does not match formal parameter\n" +
"found : string\n" +
"required: null");
}
public void testDfa11() throws Exception {
testTypes("/** @param {string} opt_x\n@return {string} */\n" +
"function f(opt_x) { if (!opt_x) { " +
"throw new Error('x cannot be empty'); } return opt_x; }");
}
public void testDfa12() throws Exception {
testTypes("/** @param {string} x \n * @constructor \n */" +
"var Bar = function(x) {};" +
"/** @param {string} x */ function g(x) { return true; }" +
"/** @param {string|number} opt_x */ " +
"function f(opt_x) { " +
" if (opt_x) { new Bar(g(opt_x) && 'x'); }" +
"}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : (number|string)\n" +
"required: string");
}
public void testDfa13() throws Exception {
testTypes(
"/**\n" +
" * @param {string} x \n" +
" * @param {number} y \n" +
" * @param {number} z \n" +
" */" +
"function g(x, y, z) {}" +
"function f() { " +
" var x = 'a'; g(x, x = 3, x);" +
"}");
}
public void testTypeInferenceWithCast1() throws Exception {
testTypes(
"/**@return {(number,null,undefined)}*/function u(x) {return null;}" +
"/**@param {number?} x\n@return {number?}*/function f(x) {return x;}" +
"/**@return {number?}*/function g(x) {" +
"var y = /**@type {number?}*/(u(x)); return f(y);}");
}
public void testTypeInferenceWithCast2() throws Exception {
testTypes(
"/**@return {(number,null,undefined)}*/function u(x) {return null;}" +
"/**@param {number?} x\n@return {number?}*/function f(x) {return x;}" +
"/**@return {number?}*/function g(x) {" +
"var y; y = /**@type {number?}*/(u(x)); return f(y);}");
}
public void testTypeInferenceWithCast3() throws Exception {
testTypes(
"/**@return {(number,null,undefined)}*/function u(x) {return 1;}" +
"/**@return {number}*/function g(x) {" +
"return /**@type {number}*/(u(x));}");
}
public void testTypeInferenceWithCast4() throws Exception {
testTypes(
"/**@return {(number,null,undefined)}*/function u(x) {return 1;}" +
"/**@return {number}*/function g(x) {" +
"return /**@type {number}*/(u(x)) && 1;}");
}
public void testTypeInferenceWithCast5() throws Exception {
testTypes(
"/** @param {number} x */ function foo(x) {}" +
"/** @param {{length:*}} y */ function bar(y) {" +
" /** @type {string} */ y.length;" +
" foo(y.length);" +
"}",
"actual parameter 1 of foo does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testTypeInferenceWithClosure1() throws Exception {
testTypes(
"/** @return {boolean} */" +
"function f() {" +
" /** @type {?string} */ var x = null;" +
" function g() { x = 'y'; } g(); " +
" return x == null;" +
"}");
}
public void testTypeInferenceWithClosure2() throws Exception {
testTypes(
"/** @return {boolean} */" +
"function f() {" +
" /** @type {?string} */ var x = null;" +
" function g() { x = 'y'; } g(); " +
" return x === 3;" +
"}",
"condition always evaluates to false\n" +
"left : (null|string)\n" +
"right: number");
}
public void testTypeInferenceWithNoEntry1() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"/** @constructor */ function Foo() {}" +
"Foo.prototype.init = function() {" +
" /** @type {?{baz: number}} */ this.bar = {baz: 3};" +
"};" +
"/**\n" +
" * @extends {Foo}\n" +
" * @constructor\n" +
" */" +
"function SubFoo() {}" +
"/** Method */" +
"SubFoo.prototype.method = function() {" +
" for (var i = 0; i < 10; i++) {" +
" f(this.bar);" +
" f(this.bar.baz);" +
" }" +
"};",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (null|{baz: number})\n" +
"required: number");
}
public void testTypeInferenceWithNoEntry2() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @param {number} x */ function f(x) {}" +
"/** @param {!Object} x */ function g(x) {}" +
"/** @constructor */ function Foo() {}" +
"Foo.prototype.init = function() {" +
" /** @type {?{baz: number}} */ this.bar = {baz: 3};" +
"};" +
"/**\n" +
" * @extends {Foo}\n" +
" * @constructor\n" +
" */" +
"function SubFoo() {}" +
"/** Method */" +
"SubFoo.prototype.method = function() {" +
" for (var i = 0; i < 10; i++) {" +
" f(this.bar);" +
" goog.asserts.assert(this.bar);" +
" g(this.bar);" +
" }" +
"};",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (null|{baz: number})\n" +
"required: number");
}
public void testForwardPropertyReference() throws Exception {
testTypes("/** @constructor */ var Foo = function() { this.init(); };" +
"/** @return {string} */" +
"Foo.prototype.getString = function() {" +
" return this.number_;" +
"};" +
"Foo.prototype.init = function() {" +
" /** @type {number} */" +
" this.number_ = 3;" +
"};",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testNoForwardTypeDeclaration() throws Exception {
testTypes(
"/** @param {MyType} x */ function f(x) {}",
"Bad type annotation. Unknown type MyType");
}
public void testNoForwardTypeDeclarationAndNoBraces() throws Exception {
testTypes("/** @return The result. */ function f() {}");
}
public void testForwardTypeDeclaration1() throws Exception {
testClosureTypes(
// malformed addDependency calls shouldn't cause a crash
"goog.addDependency();" +
"goog.addDependency('y', [goog]);" +
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/** @param {MyType} x \n * @return {number} */" +
"function f(x) { return 3; }", null);
}
public void testForwardTypeDeclaration2() throws Exception {
String f = "goog.addDependency('zzz.js', ['MyType'], []);" +
"/** @param {MyType} x */ function f(x) { }";
testClosureTypes(f, null);
testClosureTypes(f + "f(3);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: (MyType|null)");
}
public void testForwardTypeDeclaration3() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/** @param {MyType} x */ function f(x) { return x; }" +
"/** @constructor */ var MyType = function() {};" +
"f(3);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: (MyType|null)");
}
public void testForwardTypeDeclaration4() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/** @param {MyType} x */ function f(x) { return x; }" +
"/** @constructor */ var MyType = function() {};" +
"f(new MyType());",
null);
}
public void testForwardTypeDeclaration5() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/**\n" +
" * @constructor\n" +
" * @extends {MyType}\n" +
" */ var YourType = function() {};" +
"/** @override */ YourType.prototype.method = function() {};",
"Could not resolve type in @extends tag of YourType");
}
public void testForwardTypeDeclaration6() throws Exception {
testClosureTypesMultipleWarnings(
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/**\n" +
" * @constructor\n" +
" * @implements {MyType}\n" +
" */ var YourType = function() {};" +
"/** @override */ YourType.prototype.method = function() {};",
Lists.newArrayList(
"Could not resolve type in @implements tag of YourType",
"property method not defined on any superclass of YourType"));
}
public void testForwardTypeDeclaration7() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/** @param {MyType=} x */" +
"function f(x) { return x == undefined; }", null);
}
public void testForwardTypeDeclaration8() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/** @param {MyType} x */" +
"function f(x) { return x.name == undefined; }", null);
}
public void testForwardTypeDeclaration9() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/** @param {MyType} x */" +
"function f(x) { x.name = 'Bob'; }", null);
}
public void testForwardTypeDeclaration10() throws Exception {
String f = "goog.addDependency('zzz.js', ['MyType'], []);" +
"/** @param {MyType|number} x */ function f(x) { }";
testClosureTypes(f, null);
testClosureTypes(f + "f(3);", null);
testClosureTypes(f + "f('3');",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: (MyType|null|number)");
}
public void testForwardTypeDeclaration12() throws Exception {
// We assume that {Function} types can produce anything, and don't
// want to type-check them.
testClosureTypes(
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/**\n" +
" * @param {!Function} ctor\n" +
" * @return {MyType}\n" +
" */\n" +
"function f(ctor) { return new ctor(); }", null);
}
public void testForwardTypeDeclaration13() throws Exception {
// Some projects use {Function} registries to register constructors
// that aren't in their binaries. We want to make sure we can pass these
// around, but still do other checks on them.
testClosureTypes(
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/**\n" +
" * @param {!Function} ctor\n" +
" * @return {MyType}\n" +
" */\n" +
"function f(ctor) { return (new ctor()).impossibleProp; }",
"Property impossibleProp never defined on ?");
}
public void testDuplicateTypeDef() throws Exception {
testTypes(
"var goog = {};" +
"/** @constructor */ goog.Bar = function() {};" +
"/** @typedef {number} */ goog.Bar;",
"variable goog.Bar redefined with type None, " +
"original definition at [testcode]:1 " +
"with type function (new:goog.Bar): undefined");
}
public void testTypeDef1() throws Exception {
testTypes(
"var goog = {};" +
"/** @typedef {number} */ goog.Bar;" +
"/** @param {goog.Bar} x */ function f(x) {}" +
"f(3);");
}
public void testTypeDef2() throws Exception {
testTypes(
"var goog = {};" +
"/** @typedef {number} */ goog.Bar;" +
"/** @param {goog.Bar} x */ function f(x) {}" +
"f('3');",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testTypeDef3() throws Exception {
testTypes(
"var goog = {};" +
"/** @typedef {number} */ var Bar;" +
"/** @param {Bar} x */ function f(x) {}" +
"f('3');",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testTypeDef4() throws Exception {
testTypes(
"/** @constructor */ function A() {}" +
"/** @constructor */ function B() {}" +
"/** @typedef {(A|B)} */ var AB;" +
"/** @param {AB} x */ function f(x) {}" +
"f(new A()); f(new B()); f(1);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: (A|B|null)");
}
public void testTypeDef5() throws Exception {
// Notice that the error message is slightly different than
// the one for testTypeDef4, even though they should be the same.
// This is an implementation detail necessary for NamedTypes work out
// OK, and it should change if NamedTypes ever go away.
testTypes(
"/** @param {AB} x */ function f(x) {}" +
"/** @constructor */ function A() {}" +
"/** @constructor */ function B() {}" +
"/** @typedef {(A|B)} */ var AB;" +
"f(new A()); f(new B()); f(1);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: (A|B|null)");
}
public void testCircularTypeDef() throws Exception {
testTypes(
"var goog = {};" +
"/** @typedef {number|Array.<goog.Bar>} */ goog.Bar;" +
"/** @param {goog.Bar} x */ function f(x) {}" +
"f(3); f([3]); f([[3]]);");
}
public void testGetTypedPercent1() throws Exception {
String js = "var id = function(x) { return x; }\n" +
"var id2 = function(x) { return id(x); }";
assertEquals(50.0, getTypedPercent(js), 0.1);
}
public void testGetTypedPercent2() throws Exception {
String js = "var x = {}; x.y = 1;";
assertEquals(100.0, getTypedPercent(js), 0.1);
}
public void testGetTypedPercent3() throws Exception {
String js = "var f = function(x) { x.a = x.b; }";
assertEquals(50.0, getTypedPercent(js), 0.1);
}
public void testGetTypedPercent4() throws Exception {
String js = "var n = {};\n /** @constructor */ n.T = function() {};\n" +
"/** @type n.T */ var x = new n.T();";
assertEquals(100.0, getTypedPercent(js), 0.1);
}
public void testGetTypedPercent5() throws Exception {
String js = "/** @enum {number} */ keys = {A: 1,B: 2,C: 3};";
assertEquals(100.0, getTypedPercent(js), 0.1);
}
public void testGetTypedPercent6() throws Exception {
String js = "a = {TRUE: 1, FALSE: 0};";
assertEquals(100.0, getTypedPercent(js), 0.1);
}
private double getTypedPercent(String js) throws Exception {
Node n = compiler.parseTestCode(js);
Node externs = new Node(Token.BLOCK);
Node externAndJsRoot = new Node(Token.BLOCK, externs, n);
externAndJsRoot.setIsSyntheticBlock(true);
TypeCheck t = makeTypeCheck();
t.processForTesting(null, n);
return t.getTypedPercent();
}
private ObjectType getInstanceType(Node js1Node) {
JSType type = js1Node.getFirstChild().getJSType();
assertNotNull(type);
assertTrue(type instanceof FunctionType);
FunctionType functionType = (FunctionType) type;
assertTrue(functionType.isConstructor());
return functionType.getInstanceType();
}
public void testPrototypePropertyReference() throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope(""
+ "/** @constructor */\n"
+ "function Foo() {}\n"
+ "/** @param {number} a */\n"
+ "Foo.prototype.bar = function(a){};\n"
+ "/** @param {Foo} f */\n"
+ "function baz(f) {\n"
+ " Foo.prototype.bar.call(f, 3);\n"
+ "}");
assertEquals(0, compiler.getErrorCount());
assertEquals(0, compiler.getWarningCount());
assertTrue(p.scope.getVar("Foo").getType() instanceof FunctionType);
FunctionType fooType = (FunctionType) p.scope.getVar("Foo").getType();
assertEquals("function (this:Foo, number): undefined",
fooType.getPrototype().getPropertyType("bar").toString());
}
public void testResolvingNamedTypes() throws Exception {
String js = ""
+ "/** @constructor */\n"
+ "var Foo = function() {}\n"
+ "/** @param {number} a */\n"
+ "Foo.prototype.foo = function(a) {\n"
+ " return this.baz().toString();\n"
+ "};\n"
+ "/** @return {Baz} */\n"
+ "Foo.prototype.baz = function() { return new Baz(); };\n"
+ "/** @constructor\n"
+ " * @extends Foo */\n"
+ "var Bar = function() {};"
+ "/** @constructor */\n"
+ "var Baz = function() {};";
assertEquals(100.0, getTypedPercent(js), 0.1);
}
public void testMissingProperty1() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() { return this.a; };" +
"Foo.prototype.baz = function() { this.a = 3; };");
}
public void testMissingProperty2() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() { return this.a; };" +
"Foo.prototype.baz = function() { this.b = 3; };",
"Property a never defined on Foo");
}
public void testMissingProperty3() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() { return this.a; };" +
"(new Foo).a = 3;");
}
public void testMissingProperty4() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() { return this.a; };" +
"(new Foo).b = 3;",
"Property a never defined on Foo");
}
public void testMissingProperty5() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() { return this.a; };" +
"/** @constructor */ function Bar() { this.a = 3; };",
"Property a never defined on Foo");
}
public void testMissingProperty6() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() { return this.a; };" +
"/** @constructor \n * @extends {Foo} */ " +
"function Bar() { this.a = 3; };");
}
public void testMissingProperty7() throws Exception {
testTypes(
"/** @param {Object} obj */" +
"function foo(obj) { return obj.impossible; }",
"Property impossible never defined on Object");
}
public void testMissingProperty8() throws Exception {
testTypes(
"/** @param {Object} obj */" +
"function foo(obj) { return typeof obj.impossible; }");
}
public void testMissingProperty9() throws Exception {
testTypes(
"/** @param {Object} obj */" +
"function foo(obj) { if (obj.impossible) { return true; } }");
}
public void testMissingProperty10() throws Exception {
testTypes(
"/** @param {Object} obj */" +
"function foo(obj) { while (obj.impossible) { return true; } }");
}
public void testMissingProperty11() throws Exception {
testTypes(
"/** @param {Object} obj */" +
"function foo(obj) { for (;obj.impossible;) { return true; } }");
}
public void testMissingProperty12() throws Exception {
testTypes(
"/** @param {Object} obj */" +
"function foo(obj) { do { } while (obj.impossible); }");
}
public void testMissingProperty13() throws Exception {
testTypes(
"var goog = {}; goog.isDef = function(x) { return false; };" +
"/** @param {Object} obj */" +
"function foo(obj) { return goog.isDef(obj.impossible); }");
}
public void testMissingProperty14() throws Exception {
testTypes(
"var goog = {}; goog.isDef = function(x) { return false; };" +
"/** @param {Object} obj */" +
"function foo(obj) { return goog.isNull(obj.impossible); }",
"Property isNull never defined on goog");
}
public void testMissingProperty15() throws Exception {
testTypes(
"/** @param {Object} x */" +
"function f(x) { if (x.foo) { x.foo(); } }");
}
public void testMissingProperty16() throws Exception {
testTypes(
"/** @param {Object} x */" +
"function f(x) { x.foo(); if (x.foo) {} }",
"Property foo never defined on Object");
}
public void testMissingProperty17() throws Exception {
testTypes(
"/** @param {Object} x */" +
"function f(x) { if (typeof x.foo == 'function') { x.foo(); } }");
}
public void testMissingProperty18() throws Exception {
testTypes(
"/** @param {Object} x */" +
"function f(x) { if (x.foo instanceof Function) { x.foo(); } }");
}
public void testMissingProperty19() throws Exception {
testTypes(
"/** @param {Object} x */" +
"function f(x) { if (x.bar) { if (x.foo) {} } else { x.foo(); } }",
"Property foo never defined on Object");
}
public void testMissingProperty20() throws Exception {
testTypes(
"/** @param {Object} x */" +
"function f(x) { if (x.foo) { } else { x.foo(); } }",
"Property foo never defined on Object");
}
public void testMissingProperty21() throws Exception {
testTypes(
"/** @param {Object} x */" +
"function f(x) { x.foo && x.foo(); }");
}
public void testMissingProperty22() throws Exception {
testTypes(
"/** @param {Object} x \n * @return {boolean} */" +
"function f(x) { return x.foo ? x.foo() : true; }");
}
public void testMissingProperty23() throws Exception {
testTypes(
"function f(x) { x.impossible(); }",
"Property impossible never defined on x");
}
public void testMissingProperty24() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MissingType'], []);" +
"/** @param {MissingType} x */" +
"function f(x) { x.impossible(); }", null);
}
public void testMissingProperty25() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {};" +
"Foo.prototype.bar = function() {};" +
"/** @constructor */ var FooAlias = Foo;" +
"(new FooAlias()).bar();");
}
public void testMissingProperty26() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {};" +
"/** @constructor */ var FooAlias = Foo;" +
"FooAlias.prototype.bar = function() {};" +
"(new Foo()).bar();");
}
public void testMissingProperty27() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MissingType'], []);" +
"/** @param {?MissingType} x */" +
"function f(x) {" +
" for (var parent = x; parent; parent = parent.getParent()) {}" +
"}", null);
}
public void testMissingProperty28() throws Exception {
testTypes(
"function f(obj) {" +
" /** @type {*} */ obj.foo;" +
" return obj.foo;" +
"}");
testTypes(
"function f(obj) {" +
" /** @type {*} */ obj.foo;" +
" return obj.foox;" +
"}",
"Property foox never defined on obj");
}
public void testMissingProperty29() throws Exception {
// This used to emit a warning.
testTypes(
// externs
"/** @constructor */ var Foo;" +
"Foo.prototype.opera;" +
"Foo.prototype.opera.postError;",
"",
null,
false);
}
public void testMissingProperty30() throws Exception {
testTypes(
"/** @return {*} */" +
"function f() {" +
" return {};" +
"}" +
"f().a = 3;" +
"/** @param {Object} y */ function g(y) { return y.a; }");
}
public void testMissingProperty31() throws Exception {
testTypes(
"/** @return {Array|number} */" +
"function f() {" +
" return [];" +
"}" +
"f().a = 3;" +
"/** @param {Array} y */ function g(y) { return y.a; }");
}
public void testMissingProperty32() throws Exception {
testTypes(
"/** @return {Array|number} */" +
"function f() {" +
" return [];" +
"}" +
"f().a = 3;" +
"/** @param {Date} y */ function g(y) { return y.a; }",
"Property a never defined on Date");
}
public void testMissingProperty33() throws Exception {
testTypes(
"/** @param {Object} x */" +
"function f(x) { !x.foo || x.foo(); }");
}
public void testMissingProperty34() throws Exception {
testTypes(
"/** @fileoverview \n * @suppress {missingProperties} */" +
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() { return this.a; };" +
"Foo.prototype.baz = function() { this.b = 3; };");
}
public void testMissingProperty35() throws Exception {
// Bar has specialProp defined, so Bar|Baz may have specialProp defined.
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @constructor */ function Bar() {}" +
"/** @constructor */ function Baz() {}" +
"/** @param {Foo|Bar} x */ function f(x) { x.specialProp = 1; }" +
"/** @param {Bar|Baz} x */ function g(x) { return x.specialProp; }");
}
public void testMissingProperty36() throws Exception {
// Foo has baz defined, and SubFoo has bar defined, so some objects with
// bar may have baz.
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.baz = 0;" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"SubFoo.prototype.bar = 0;" +
"/** @param {{bar: number}} x */ function f(x) { return x.baz; }");
}
public void testMissingProperty37() throws Exception {
// This used to emit a missing property warning because we couldn't
// determine that the inf(Foo, {isVisible:boolean}) == SubFoo.
testTypes(
"/** @param {{isVisible: boolean}} x */ function f(x){" +
" x.isVisible = false;" +
"}" +
"/** @constructor */ function Foo() {}" +
"/**\n" +
" * @constructor \n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/** @type {boolean} */ SubFoo.prototype.isVisible = true;" +
"/**\n" +
" * @param {Foo} x\n" +
" * @return {boolean}\n" +
" */\n" +
"function g(x) { return x.isVisible; }");
}
public void testMissingProperty38() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @constructor */ function Bar() {}" +
"/** @return {Foo|Bar} */ function f() { return new Foo(); }" +
"f().missing;",
"Property missing never defined on (Bar|Foo|null)");
}
public void testMissingProperty39() throws Exception {
testTypes(
"/** @return {string|number} */ function f() { return 3; }" +
"f().length;");
}
public void testMissingProperty40() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MissingType'], []);" +
"/** @param {(Array|MissingType)} x */" +
"function f(x) { x.impossible(); }", null);
}
public void testMissingProperty41() throws Exception {
testTypes(
"/** @param {(Array|Date)} x */" +
"function f(x) { if (x.impossible) x.impossible(); }");
}
public void testMissingProperty42() throws Exception {
testTypes(
"/** @param {Object} x */" +
"function f(x) { " +
" if (typeof x.impossible == 'undefined') throw Error();" +
" return x.impossible;" +
"}");
}
public void testReflectObject1() throws Exception {
testClosureTypes(
"var goog = {}; goog.reflect = {}; " +
"goog.reflect.object = function(x, y){};" +
"/** @constructor */ function A() {}" +
"goog.reflect.object(A, {x: 3});",
null);
}
public void testReflectObject2() throws Exception {
testClosureTypes(
"var goog = {}; goog.reflect = {}; " +
"goog.reflect.object = function(x, y){};" +
"/** @param {string} x */ function f(x) {}" +
"/** @constructor */ function A() {}" +
"goog.reflect.object(A, {x: f(1 + 1)});",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testLends1() throws Exception {
testTypes(
"function extend(x, y) {}" +
"/** @constructor */ function Foo() {}" +
"extend(Foo, /** @lends */ ({bar: 1}));",
"Bad type annotation. missing object name in @lends tag");
}
public void testLends2() throws Exception {
testTypes(
"function extend(x, y) {}" +
"/** @constructor */ function Foo() {}" +
"extend(Foo, /** @lends {Foob} */ ({bar: 1}));",
"Variable Foob not declared before @lends annotation.");
}
public void testLends3() throws Exception {
testTypes(
"function extend(x, y) {}" +
"/** @constructor */ function Foo() {}" +
"extend(Foo, {bar: 1});" +
"alert(Foo.bar);",
"Property bar never defined on Foo");
}
public void testLends4() throws Exception {
testTypes(
"function extend(x, y) {}" +
"/** @constructor */ function Foo() {}" +
"extend(Foo, /** @lends {Foo} */ ({bar: 1}));" +
"alert(Foo.bar);");
}
public void testLends5() throws Exception {
testTypes(
"function extend(x, y) {}" +
"/** @constructor */ function Foo() {}" +
"extend(Foo, {bar: 1});" +
"alert((new Foo()).bar);",
"Property bar never defined on Foo");
}
public void testLends6() throws Exception {
testTypes(
"function extend(x, y) {}" +
"/** @constructor */ function Foo() {}" +
"extend(Foo, /** @lends {Foo.prototype} */ ({bar: 1}));" +
"alert((new Foo()).bar);");
}
public void testLends7() throws Exception {
testTypes(
"function extend(x, y) {}" +
"/** @constructor */ function Foo() {}" +
"extend(Foo, /** @lends {Foo.prototype|Foo} */ ({bar: 1}));",
"Bad type annotation. expected closing }");
}
public void testLends8() throws Exception {
testTypes(
"function extend(x, y) {}" +
"/** @type {number} */ var Foo = 3;" +
"extend(Foo, /** @lends {Foo} */ ({bar: 1}));",
"May only lend properties to object types. Foo has type number.");
}
public void testLends9() throws Exception {
testClosureTypesMultipleWarnings(
"function extend(x, y) {}" +
"/** @constructor */ function Foo() {}" +
"extend(Foo, /** @lends {!Foo} */ ({bar: 1}));",
Lists.newArrayList(
"Bad type annotation. expected closing }",
"Bad type annotation. missing object name in @lends tag"));
}
public void testLends10() throws Exception {
testTypes(
"function defineClass(x) { return function() {}; } " +
"/** @constructor */" +
"var Foo = defineClass(" +
" /** @lends {Foo.prototype} */ ({/** @type {number} */ bar: 1}));" +
"/** @return {string} */ function f() { return (new Foo()).bar; }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testLends11() throws Exception {
testTypes(
"function defineClass(x, y) { return function() {}; } " +
"/** @constructor */" +
"var Foo = function() {};" +
"/** @return {*} */ Foo.prototype.bar = function() { return 3; };" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */\n" +
"var SubFoo = defineClass(Foo, " +
" /** @lends {SubFoo.prototype} */ ({\n" +
" /** @return {number} */ bar: function() { return 3; }}));" +
"/** @return {string} */ function f() { return (new SubFoo()).bar(); }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testDeclaredNativeTypeEquality() throws Exception {
Node n = parseAndTypeCheck("/** @constructor */ function Object() {};");
assertTypeEquals(registry.getNativeType(JSTypeNative.OBJECT_FUNCTION_TYPE),
n.getFirstChild().getJSType());
}
public void testUndefinedVar() throws Exception {
Node n = parseAndTypeCheck("var undefined;");
assertTypeEquals(registry.getNativeType(JSTypeNative.VOID_TYPE),
n.getFirstChild().getFirstChild().getJSType());
}
public void testFlowScopeBug1() throws Exception {
Node n = parseAndTypeCheck("/** @param {number} a \n"
+ "* @param {number} b */\n"
+ "function f(a, b) {\n"
+ "/** @type number */"
+ "var i = 0;"
+ "for (; (i + a) < b; ++i) {}}");
// check the type of the add node for i + f
assertTypeEquals(registry.getNativeType(JSTypeNative.NUMBER_TYPE),
n.getFirstChild().getLastChild().getLastChild().getFirstChild()
.getNext().getFirstChild().getJSType());
}
public void testFlowScopeBug2() throws Exception {
Node n = parseAndTypeCheck("/** @constructor */ function Foo() {};\n"
+ "Foo.prototype.hi = false;"
+ "function foo(a, b) {\n"
+ " /** @type Array */"
+ " var arr;"
+ " /** @type number */"
+ " var iter;"
+ " for (iter = 0; iter < arr.length; ++ iter) {"
+ " /** @type Foo */"
+ " var afoo = arr[iter];"
+ " afoo;"
+ " }"
+ "}");
// check the type of afoo when referenced
assertTypeEquals(registry.createNullableType(registry.getType("Foo")),
n.getLastChild().getLastChild().getLastChild().getLastChild()
.getLastChild().getLastChild().getJSType());
}
public void testAddSingletonGetter() {
Node n = parseAndTypeCheck(
"/** @constructor */ function Foo() {};\n" +
"goog.addSingletonGetter(Foo);");
ObjectType o = (ObjectType) n.getFirstChild().getJSType();
assertEquals("function (): Foo",
o.getPropertyType("getInstance").toString());
assertEquals("Foo", o.getPropertyType("instance_").toString());
}
public void testTypeCheckStandaloneAST() throws Exception {
Node n = compiler.parseTestCode("function Foo() { }");
typeCheck(n);
MemoizedScopeCreator scopeCreator = new MemoizedScopeCreator(
new TypedScopeCreator(compiler));
Scope topScope = scopeCreator.createScope(n, null);
Node second = compiler.parseTestCode("new Foo");
Node externs = new Node(Token.BLOCK);
Node externAndJsRoot = new Node(Token.BLOCK, externs, second);
externAndJsRoot.setIsSyntheticBlock(true);
new TypeCheck(
compiler,
new SemanticReverseAbstractInterpreter(
compiler.getCodingConvention(), registry),
registry, topScope, scopeCreator, CheckLevel.WARNING, CheckLevel.OFF)
.process(null, second);
assertEquals(1, compiler.getWarningCount());
assertEquals("cannot instantiate non-constructor",
compiler.getWarnings()[0].description);
}
public void testUpdateParameterTypeOnClosure() throws Exception {
testTypes(
"/**\n" +
"* @constructor\n" +
"* @param {*=} opt_value\n" +
"* @return {?}\n" +
"*/\n" +
"function Object(opt_value) {}\n" +
"/**\n" +
"* @constructor\n" +
"* @param {...*} var_args\n" +
"*/\n" +
"function Function(var_args) {}\n" +
"/**\n" +
"* @type {Function}\n" +
"*/\n" +
// The line below sets JSDocInfo on Object so that the type of the
// argument to function f has JSDoc through its prototype chain.
"Object.prototype.constructor = function() {};\n",
"/**\n" +
"* @param {function(): boolean} fn\n" +
"*/\n" +
"function f(fn) {}\n" +
"f(function(g) { });\n",
null,
false);
}
public void testTemplatedThisType1() throws Exception {
testTypes(
"/** @constructor */\n" +
"function Foo() {}\n" +
"/**\n" +
" * @this {T}\n" +
" * @return {T}\n" +
" * @template T\n" +
" */\n" +
"Foo.prototype.method = function() {};\n" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */\n" +
"function Bar() {}\n" +
"var g = new Bar().method();\n" +
"/**\n" +
" * @param {number} a\n" +
" */\n" +
"function compute(a) {};\n" +
"compute(g);\n",
"actual parameter 1 of compute does not match formal parameter\n" +
"found : Bar\n" +
"required: number");
}
public void testTemplatedThisType2() throws Exception {
testTypes(
"/**\n" +
" * @this {Array.<T>|{length:number}}\n" +
" * @return {T}\n" +
" * @template T\n" +
" */\n" +
"Array.prototype.method = function() {};\n" +
"(function(){\n" +
" Array.prototype.method.call(arguments);" +
"})();");
}
public void testTemplateType1() throws Exception {
testTypes(
"/**\n" +
"* @param {T} x\n" +
"* @param {T} y\n" +
"* @param {function(this:T, ...)} z\n" +
"* @template T\n" +
"*/\n" +
"function f(x, y, z) {}\n" +
"f(this, this, function() { this });");
}
public void testTemplateType2() throws Exception {
// "this" types need to be coerced for ES3 style function or left
// allow for ES5-strict methods.
testTypes(
"/**\n" +
"* @param {T} x\n" +
"* @param {function(this:T, ...)} y\n" +
"* @template T\n" +
"*/\n" +
"function f(x, y) {}\n" +
"f(0, function() {});");
}
public void testTemplateType3() throws Exception {
testTypes(
"/**" +
" * @param {T} v\n" +
" * @param {function(T)} f\n" +
" * @template T\n" +
" */\n" +
"function call(v, f) { f.call(null, v); }" +
"/** @type {string} */ var s;" +
"call(3, function(x) {" +
" x = true;" +
" s = x;" +
"});",
"assignment\n" +
"found : boolean\n" +
"required: string");
}
public void testTemplateType4() throws Exception {
testTypes(
"/**" +
" * @param {...T} p\n" +
" * @return {T} \n" +
" * @template T\n" +
" */\n" +
"function fn(p) { return p; }\n" +
"/** @type {!Object} */ var x;" +
"x = fn(3, null);",
"assignment\n" +
"found : (null|number)\n" +
"required: Object");
}
public void testTemplateType5() throws Exception {
testTypes(
"/**" +
" * @param {Array.<T>} arr \n" +
" * @param {?function(T)} f \n" +
" * @return {T} \n" +
" * @template T\n" +
" */\n" +
"function fn(arr, f) { return arr[0]; }\n" +
"/** @param {Array.<number>} arr */ function g(arr) {" +
" /** @type {!Object} */ var x = fn.call(null, arr, null);" +
"}",
"initializing variable\n" +
"found : number\n" +
"required: Object");
}
public void disable_testBadTemplateType4() throws Exception {
// TODO(johnlenz): Add a check for useless of template types.
// Unless there are at least two references to a Template type in
// a definition it isn't useful.
testTypes(
"/**\n" +
"* @template T\n" +
"*/\n" +
"function f() {}\n" +
"f();",
FunctionTypeBuilder.TEMPLATE_TYPE_EXPECTED.format());
}
public void disable_testBadTemplateType5() throws Exception {
// TODO(johnlenz): Add a check for useless of template types.
// Unless there are at least two references to a Template type in
// a definition it isn't useful.
testTypes(
"/**\n" +
"* @template T\n" +
"* @return {T}\n" +
"*/\n" +
"function f() {}\n" +
"f();",
FunctionTypeBuilder.TEMPLATE_TYPE_EXPECTED.format());
}
public void disable_testFunctionLiteralUndefinedThisArgument()
throws Exception {
// TODO(johnlenz): this was a weird error. We should add a general
// restriction on what is accepted for T. Something like:
// "@template T of {Object|string}" or some such.
testTypes(""
+ "/**\n"
+ " * @param {function(this:T, ...)?} fn\n"
+ " * @param {?T} opt_obj\n"
+ " * @template T\n"
+ " */\n"
+ "function baz(fn, opt_obj) {}\n"
+ "baz(function() { this; });",
"Function literal argument refers to undefined this argument");
}
public void testFunctionLiteralDefinedThisArgument() throws Exception {
testTypes(""
+ "/**\n"
+ " * @param {function(this:T, ...)?} fn\n"
+ " * @param {?T} opt_obj\n"
+ " * @template T\n"
+ " */\n"
+ "function baz(fn, opt_obj) {}\n"
+ "baz(function() { this; }, {});");
}
public void testFunctionLiteralDefinedThisArgument2() throws Exception {
testTypes(""
+ "/** @param {string} x */ function f(x) {}"
+ "/**\n"
+ " * @param {?function(this:T, ...)} fn\n"
+ " * @param {T=} opt_obj\n"
+ " * @template T\n"
+ " */\n"
+ "function baz(fn, opt_obj) {}\n"
+ "function g() { baz(function() { f(this.length); }, []); }",
"actual parameter 1 of f does not match formal parameter\n"
+ "found : number\n"
+ "required: string");
}
public void testFunctionLiteralUnreadNullThisArgument() throws Exception {
testTypes(""
+ "/**\n"
+ " * @param {function(this:T, ...)?} fn\n"
+ " * @param {?T} opt_obj\n"
+ " * @template T\n"
+ " */\n"
+ "function baz(fn, opt_obj) {}\n"
+ "baz(function() {}, null);");
}
public void testUnionTemplateThisType() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** @return {F|Array} */ function g() { return []; }" +
"/** @param {F} x */ function h(x) { }" +
"/**\n" +
"* @param {T} x\n" +
"* @param {function(this:T, ...)} y\n" +
"* @template T\n" +
"*/\n" +
"function f(x, y) {}\n" +
"f(g(), function() { h(this); });",
"actual parameter 1 of h does not match formal parameter\n" +
"found : (Array|F|null)\n" +
"required: (F|null)");
}
public void testActiveXObject() throws Exception {
testTypes(
"/** @type {Object} */ var x = new ActiveXObject();" +
"/** @type { {impossibleProperty} } */ var y = new ActiveXObject();");
}
public void testRecordType1() throws Exception {
testTypes(
"/** @param {{prop: number}} x */" +
"function f(x) {}" +
"f({});",
"actual parameter 1 of f does not match formal parameter\n" +
"found : {prop: (number|undefined)}\n" +
"required: {prop: number}");
}
public void testRecordType2() throws Exception {
testTypes(
"/** @param {{prop: (number|undefined)}} x */" +
"function f(x) {}" +
"f({});");
}
public void testRecordType3() throws Exception {
testTypes(
"/** @param {{prop: number}} x */" +
"function f(x) {}" +
"f({prop: 'x'});",
"actual parameter 1 of f does not match formal parameter\n" +
"found : {prop: (number|string)}\n" +
"required: {prop: number}");
}
public void testRecordType4() throws Exception {
// Notice that we do not do flow-based inference on the object type:
// We don't try to prove that x.prop may not be string until x
// gets passed to g.
testClosureTypesMultipleWarnings(
"/** @param {{prop: (number|undefined)}} x */" +
"function f(x) {}" +
"/** @param {{prop: (string|undefined)}} x */" +
"function g(x) {}" +
"var x = {}; f(x); g(x);",
Lists.newArrayList(
"actual parameter 1 of f does not match formal parameter\n" +
"found : {prop: (number|string|undefined)}\n" +
"required: {prop: (number|undefined)}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : {prop: (number|string|undefined)}\n" +
"required: {prop: (string|undefined)}"));
}
public void testRecordType5() throws Exception {
testTypes(
"/** @param {{prop: (number|undefined)}} x */" +
"function f(x) {}" +
"/** @param {{otherProp: (string|undefined)}} x */" +
"function g(x) {}" +
"var x = {}; f(x); g(x);");
}
public void testRecordType6() throws Exception {
testTypes(
"/** @return {{prop: (number|undefined)}} x */" +
"function f() { return {}; }");
}
public void testRecordType7() throws Exception {
testTypes(
"/** @return {{prop: (number|undefined)}} x */" +
"function f() { var x = {}; g(x); return x; }" +
"/** @param {number} x */" +
"function g(x) {}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : {prop: (number|undefined)}\n" +
"required: number");
}
public void testRecordType8() throws Exception {
testTypes(
"/** @return {{prop: (number|string)}} x */" +
"function f() { var x = {prop: 3}; g(x.prop); return x; }" +
"/** @param {string} x */" +
"function g(x) {}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testDuplicateRecordFields1() throws Exception {
testTypes("/**"
+ "* @param {{x:string, x:number}} a"
+ "*/"
+ "function f(a) {};",
"Parse error. Duplicate record field x");
}
public void testDuplicateRecordFields2() throws Exception {
testTypes("/**"
+ "* @param {{name:string,number:x,number:y}} a"
+ " */"
+ "function f(a) {};",
new String[] {"Bad type annotation. Unknown type x",
"Parse error. Duplicate record field number",
"Bad type annotation. Unknown type y"});
}
public void testMultipleExtendsInterface1() throws Exception {
testTypes("/** @interface */ function base1() {}\n"
+ "/** @interface */ function base2() {}\n"
+ "/** @interface\n"
+ "* @extends {base1}\n"
+ "* @extends {base2}\n"
+ "*/\n"
+ "function derived() {}");
}
public void testMultipleExtendsInterface2() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @desc description */Int0.prototype.foo = function() {};" +
"/** @interface \n @extends {Int0} \n @extends {Int1} */" +
"function Int2() {};" +
"/** @constructor\n @implements {Int2} */function Foo() {};",
"property foo on interface Int0 is not implemented by type Foo");
}
public void testMultipleExtendsInterface3() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @desc description */Int1.prototype.foo = function() {};" +
"/** @interface \n @extends {Int0} \n @extends {Int1} */" +
"function Int2() {};" +
"/** @constructor\n @implements {Int2} */function Foo() {};",
"property foo on interface Int1 is not implemented by type Foo");
}
public void testMultipleExtendsInterface4() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @interface \n @extends {Int0} \n @extends {Int1} \n" +
" @extends {number} */" +
"function Int2() {};" +
"/** @constructor\n @implements {Int2} */function Foo() {};",
"Int2 @extends non-object type number");
}
public void testMultipleExtendsInterface5() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @constructor */function Int1() {};" +
"/** @desc description @ return {string} x */" +
"/** @interface \n @extends {Int0} \n @extends {Int1} */" +
"function Int2() {};",
"Int2 cannot extend this type; interfaces can only extend interfaces");
}
public void testMultipleExtendsInterface6() throws Exception {
testTypes(
"/** @interface */function Super1() {};" +
"/** @interface */function Super2() {};" +
"/** @param {number} bar */Super2.prototype.foo = function(bar) {};" +
"/** @interface\n @extends {Super1}\n " +
"@extends {Super2} */function Sub() {};" +
"/** @override\n @param {string} bar */Sub.prototype.foo =\n" +
"function(bar) {};",
"mismatch of the foo property type and the type of the property it " +
"overrides from superclass Super2\n" +
"original: function (this:Super2, number): undefined\n" +
"override: function (this:Sub, string): undefined");
}
public void testMultipleExtendsInterfaceAssignment() throws Exception {
testTypes("/** @interface */var I1 = function() {};\n" +
"/** @interface */ var I2 = function() {}\n" +
"/** @interface\n@extends {I1}\n@extends {I2}*/" +
"var I3 = function() {};\n" +
"/** @constructor\n@implements {I3}*/var T = function() {};\n" +
"var t = new T();\n" +
"/** @type {I1} */var i1 = t;\n" +
"/** @type {I2} */var i2 = t;\n" +
"/** @type {I3} */var i3 = t;\n" +
"i1 = i3;\n" +
"i2 = i3;\n");
}
public void testMultipleExtendsInterfaceParamPass() throws Exception {
testTypes("/** @interface */var I1 = function() {};\n" +
"/** @interface */ var I2 = function() {}\n" +
"/** @interface\n@extends {I1}\n@extends {I2}*/" +
"var I3 = function() {};\n" +
"/** @constructor\n@implements {I3}*/var T = function() {};\n" +
"var t = new T();\n" +
"/** @param x I1 \n@param y I2\n@param z I3*/function foo(x,y,z){};\n" +
"foo(t,t,t)\n");
}
public void testBadMultipleExtendsClass() throws Exception {
testTypes("/** @constructor */ function base1() {}\n"
+ "/** @constructor */ function base2() {}\n"
+ "/** @constructor\n"
+ "* @extends {base1}\n"
+ "* @extends {base2}\n"
+ "*/\n"
+ "function derived() {}",
"Bad type annotation. type annotation incompatible "
+ "with other annotations");
}
public void testInterfaceExtendsResolution() throws Exception {
testTypes("/** @interface \n @extends {A} */ function B() {};\n" +
"/** @constructor \n @implements {B} */ function C() {};\n" +
"/** @interface */ function A() {};");
}
public void testPropertyCanBeDefinedInObject() throws Exception {
testTypes("/** @interface */ function I() {};" +
"I.prototype.bar = function() {};" +
"/** @type {Object} */ var foo;" +
"foo.bar();");
}
private void checkObjectType(ObjectType objectType, String propertyName,
JSType expectedType) {
assertTrue("Expected " + objectType.getReferenceName() +
" to have property " +
propertyName, objectType.hasProperty(propertyName));
assertTypeEquals("Expected " + objectType.getReferenceName() +
"'s property " +
propertyName + " to have type " + expectedType,
expectedType, objectType.getPropertyType(propertyName));
}
public void testExtendedInterfacePropertiesCompatibility1() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @type {number} */" +
"Int0.prototype.foo;" +
"/** @type {string} */" +
"Int1.prototype.foo;" +
"/** @interface \n @extends {Int0} \n @extends {Int1} */" +
"function Int2() {};",
"Interface Int2 has a property foo with incompatible types in its " +
"super interfaces Int0 and Int1");
}
public void testExtendedInterfacePropertiesCompatibility2() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @interface */function Int2() {};" +
"/** @type {number} */" +
"Int0.prototype.foo;" +
"/** @type {string} */" +
"Int1.prototype.foo;" +
"/** @type {Object} */" +
"Int2.prototype.foo;" +
"/** @interface \n @extends {Int0} \n @extends {Int1} \n" +
"@extends {Int2}*/" +
"function Int3() {};",
new String[] {
"Interface Int3 has a property foo with incompatible types in " +
"its super interfaces Int0 and Int1",
"Interface Int3 has a property foo with incompatible types in " +
"its super interfaces Int1 and Int2"
});
}
public void testExtendedInterfacePropertiesCompatibility3() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @type {number} */" +
"Int0.prototype.foo;" +
"/** @type {string} */" +
"Int1.prototype.foo;" +
"/** @interface \n @extends {Int1} */ function Int2() {};" +
"/** @interface \n @extends {Int0} \n @extends {Int2} */" +
"function Int3() {};",
"Interface Int3 has a property foo with incompatible types in its " +
"super interfaces Int0 and Int1");
}
public void testExtendedInterfacePropertiesCompatibility4() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface \n @extends {Int0} */ function Int1() {};" +
"/** @type {number} */" +
"Int0.prototype.foo;" +
"/** @interface */function Int2() {};" +
"/** @interface \n @extends {Int2} */ function Int3() {};" +
"/** @type {string} */" +
"Int2.prototype.foo;" +
"/** @interface \n @extends {Int1} \n @extends {Int3} */" +
"function Int4() {};",
"Interface Int4 has a property foo with incompatible types in its " +
"super interfaces Int0 and Int2");
}
public void testExtendedInterfacePropertiesCompatibility5() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @type {number} */" +
"Int0.prototype.foo;" +
"/** @type {string} */" +
"Int1.prototype.foo;" +
"/** @interface \n @extends {Int1} */ function Int2() {};" +
"/** @interface \n @extends {Int0} \n @extends {Int2} */" +
"function Int3() {};" +
"/** @interface */function Int4() {};" +
"/** @type {number} */" +
"Int4.prototype.foo;" +
"/** @interface \n @extends {Int3} \n @extends {Int4} */" +
"function Int5() {};",
new String[] {
"Interface Int3 has a property foo with incompatible types in its" +
" super interfaces Int0 and Int1",
"Interface Int5 has a property foo with incompatible types in its" +
" super interfaces Int1 and Int4"});
}
public void testExtendedInterfacePropertiesCompatibility6() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @type {number} */" +
"Int0.prototype.foo;" +
"/** @type {string} */" +
"Int1.prototype.foo;" +
"/** @interface \n @extends {Int1} */ function Int2() {};" +
"/** @interface \n @extends {Int0} \n @extends {Int2} */" +
"function Int3() {};" +
"/** @interface */function Int4() {};" +
"/** @type {string} */" +
"Int4.prototype.foo;" +
"/** @interface \n @extends {Int3} \n @extends {Int4} */" +
"function Int5() {};",
"Interface Int3 has a property foo with incompatible types in its" +
" super interfaces Int0 and Int1");
}
public void testExtendedInterfacePropertiesCompatibility7() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @type {number} */" +
"Int0.prototype.foo;" +
"/** @type {string} */" +
"Int1.prototype.foo;" +
"/** @interface \n @extends {Int1} */ function Int2() {};" +
"/** @interface \n @extends {Int0} \n @extends {Int2} */" +
"function Int3() {};" +
"/** @interface */function Int4() {};" +
"/** @type {Object} */" +
"Int4.prototype.foo;" +
"/** @interface \n @extends {Int3} \n @extends {Int4} */" +
"function Int5() {};",
new String[] {
"Interface Int3 has a property foo with incompatible types in its" +
" super interfaces Int0 and Int1",
"Interface Int5 has a property foo with incompatible types in its" +
" super interfaces Int1 and Int4"});
}
public void testExtendedInterfacePropertiesCompatibility8() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @type {number} */" +
"Int0.prototype.foo;" +
"/** @type {string} */" +
"Int1.prototype.bar;" +
"/** @interface \n @extends {Int1} */ function Int2() {};" +
"/** @interface \n @extends {Int0} \n @extends {Int2} */" +
"function Int3() {};" +
"/** @interface */function Int4() {};" +
"/** @type {Object} */" +
"Int4.prototype.foo;" +
"/** @type {Null} */" +
"Int4.prototype.bar;" +
"/** @interface \n @extends {Int3} \n @extends {Int4} */" +
"function Int5() {};",
new String[] {
"Interface Int5 has a property bar with incompatible types in its" +
" super interfaces Int1 and Int4",
"Interface Int5 has a property foo with incompatible types in its" +
" super interfaces Int0 and Int4"});
}
public void testGenerics1() throws Exception {
String FN_DECL = "/** \n" +
" * @param {T} x \n" +
" * @param {function(T):T} y \n" +
" * @template T\n" +
" */ \n" +
"function f(x,y) { return y(x); }\n";
testTypes(
FN_DECL +
"/** @type {string} */" +
"var out;" +
"/** @type {string} */" +
"var result = f('hi', function(x){ out = x; return x; });");
testTypes(
FN_DECL +
"/** @type {string} */" +
"var out;" +
"var result = f(0, function(x){ out = x; return x; });",
"assignment\n" +
"found : number\n" +
"required: string");
testTypes(
FN_DECL +
"var out;" +
"/** @type {string} */" +
"var result = f(0, function(x){ out = x; return x; });",
"assignment\n" +
"found : number\n" +
"required: string");
}
public void testFilter0()
throws Exception {
testTypes(
"/**\n" +
" * @param {T} arr\n" +
" * @return {T}\n" +
" * @template T\n" +
" */\n" +
"var filter = function(arr){};\n" +
"/** @type {!Array.<string>} */" +
"var arr;\n" +
"/** @type {!Array.<string>} */" +
"var result = filter(arr);");
}
public void testFilter1()
throws Exception {
testTypes(
"/**\n" +
" * @param {!Array.<T>} arr\n" +
" * @return {!Array.<T>}\n" +
" * @template T\n" +
" */\n" +
"var filter = function(arr){};\n" +
"/** @type {!Array.<string>} */" +
"var arr;\n" +
"/** @type {!Array.<string>} */" +
"var result = filter(arr);");
}
public void testFilter2()
throws Exception {
testTypes(
"/**\n" +
" * @param {!Array.<T>} arr\n" +
" * @return {!Array.<T>}\n" +
" * @template T\n" +
" */\n" +
"var filter = function(arr){};\n" +
"/** @type {!Array.<string>} */" +
"var arr;\n" +
"/** @type {!Array.<number>} */" +
"var result = filter(arr);",
"initializing variable\n" +
"found : Array.<string>\n" +
"required: Array.<number>");
}
public void testFilter3()
throws Exception {
testTypes(
"/**\n" +
" * @param {Array.<T>} arr\n" +
" * @return {Array.<T>}\n" +
" * @template T\n" +
" */\n" +
"var filter = function(arr){};\n" +
"/** @type {Array.<string>} */" +
"var arr;\n" +
"/** @type {Array.<number>} */" +
"var result = filter(arr);",
"initializing variable\n" +
"found : (Array.<string>|null)\n" +
"required: (Array.<number>|null)");
}
public void testBackwardsInferenceGoogArrayFilter1()
throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {Array.<string>} */" +
"var arr;\n" +
"/** @type {!Array.<number>} */" +
"var result = goog.array.filter(" +
" arr," +
" function(item,index,src) {return false;});",
"initializing variable\n" +
"found : Array.<string>\n" +
"required: Array.<number>");
}
public void testBackwardsInferenceGoogArrayFilter2() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {number} */" +
"var out;" +
"/** @type {Array.<string>} */" +
"var arr;\n" +
"var out4 = goog.array.filter(" +
" arr," +
" function(item,index,src) {out = item;});",
"assignment\n" +
"found : string\n" +
"required: number");
}
public void testBackwardsInferenceGoogArrayFilter3() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {string} */" +
"var out;" +
"/** @type {Array.<string>} */ var arr;\n" +
"var result = goog.array.filter(" +
" arr," +
" function(item,index,src) {out = index;});",
"assignment\n" +
"found : number\n" +
"required: string");
}
public void testBackwardsInferenceGoogArrayFilter4() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {string} */" +
"var out;" +
"/** @type {Array.<string>} */ var arr;\n" +
"var out4 = goog.array.filter(" +
" arr," +
" function(item,index,srcArr) {out = srcArr;});",
"assignment\n" +
"found : (null|{length: number})\n" +
"required: string");
}
public void testCatchExpression1() throws Exception {
testTypes(
"function fn() {" +
" /** @type {number} */" +
" var out = 0;" +
" try {\n" +
" foo();\n" +
" } catch (/** @type {string} */ e) {\n" +
" out = e;" +
" }" +
"}\n",
"assignment\n" +
"found : string\n" +
"required: number");
}
public void testCatchExpression2() throws Exception {
testTypes(
"function fn() {" +
" /** @type {number} */" +
" var out = 0;" +
" /** @type {string} */" +
" var e;" +
" try {\n" +
" foo();\n" +
" } catch (e) {\n" +
" out = e;" +
" }" +
"}\n");
}
public void testParameterized1() throws Exception {
testTypes(
"/** @type {!Array.<string>} */" +
"var arr1 = [];\n" +
"/** @type {!Array.<number>} */" +
"var arr2 = [];\n" +
"arr1 = arr2;",
"assignment\n" +
"found : Array.<number>\n" +
"required: Array.<string>");
}
public void testParameterized2() throws Exception {
testTypes(
"/** @type {!Array.<string>} */" +
"var arr1 = /** @type {!Array.<number>} */([]);\n",
"initializing variable\n" +
"found : Array.<number>\n" +
"required: Array.<string>");
}
public void testParameterized3() throws Exception {
testTypes(
"/** @type {Array.<string>} */" +
"var arr1 = /** @type {!Array.<number>} */([]);\n",
"initializing variable\n" +
"found : Array.<number>\n" +
"required: (Array.<string>|null)");
}
public void testParameterized4() throws Exception {
testTypes(
"/** @type {Array.<string>} */" +
"var arr1 = [];\n" +
"/** @type {Array.<number>} */" +
"var arr2 = arr1;\n",
"initializing variable\n" +
"found : (Array.<string>|null)\n" +
"required: (Array.<number>|null)");
}
public void testParameterizedTypeSubtypes2() throws Exception {
JSType arrayOfNumber = createParameterizedType(
ARRAY_TYPE, NUMBER_TYPE);
JSType arrayOfString = createParameterizedType(
ARRAY_TYPE, STRING_TYPE);
assertFalse(arrayOfString.isSubtype(createUnionType(arrayOfNumber, NULL_VOID)));
}
private void testTypes(String js) throws Exception {
testTypes(js, (String) null);
}
private void testTypes(String js, String description) throws Exception {
testTypes(js, description, false);
}
private void testTypes(String js, DiagnosticType type) throws Exception {
testTypes(js, type.format(), false);
}
private void testClosureTypes(String js, String description)
throws Exception {
testClosureTypesMultipleWarnings(js,
description == null ? null : Lists.newArrayList(description));
}
private void testClosureTypesMultipleWarnings(
String js, List<String> descriptions) throws Exception {
Node n = compiler.parseTestCode(js);
Node externs = new Node(Token.BLOCK);
Node externAndJsRoot = new Node(Token.BLOCK, externs, n);
externAndJsRoot.setIsSyntheticBlock(true);
assertEquals("parsing error: " +
Joiner.on(", ").join(compiler.getErrors()),
0, compiler.getErrorCount());
// For processing goog.addDependency for forward typedefs.
new ProcessClosurePrimitives(compiler, null, CheckLevel.ERROR)
.process(null, n);
CodingConvention convention = compiler.getCodingConvention();
new TypeCheck(compiler,
new ClosureReverseAbstractInterpreter(
convention, registry).append(
new SemanticReverseAbstractInterpreter(
convention, registry))
.getFirst(),
registry)
.processForTesting(null, n);
assertEquals(
"unexpected error(s) : " +
Joiner.on(", ").join(compiler.getErrors()),
0, compiler.getErrorCount());
if (descriptions == null) {
assertEquals(
"unexpected warning(s) : " +
Joiner.on(", ").join(compiler.getWarnings()),
0, compiler.getWarningCount());
} else {
assertEquals(
"unexpected warning(s) : " +
Joiner.on(", ").join(compiler.getWarnings()),
descriptions.size(), compiler.getWarningCount());
Set<String> actualWarningDescriptions = Sets.newHashSet();
for (int i = 0; i < descriptions.size(); i++) {
actualWarningDescriptions.add(compiler.getWarnings()[i].description);
}
assertEquals(
Sets.newHashSet(descriptions), actualWarningDescriptions);
}
}
void testTypes(String js, String description, boolean isError)
throws Exception {
testTypes(DEFAULT_EXTERNS, js, description, isError);
}
void testTypes(String externs, String js, String description, boolean isError)
throws Exception {
Node n = parseAndTypeCheck(externs, js);
JSError[] errors = compiler.getErrors();
if (description != null && isError) {
assertTrue("expected an error", errors.length > 0);
assertEquals(description, errors[0].description);
errors = Arrays.asList(errors).subList(1, errors.length).toArray(
new JSError[errors.length - 1]);
}
if (errors.length > 0) {
fail("unexpected error(s):\n" + Joiner.on("\n").join(errors));
}
JSError[] warnings = compiler.getWarnings();
if (description != null && !isError) {
assertTrue("expected a warning", warnings.length > 0);
assertEquals(description, warnings[0].description);
warnings = Arrays.asList(warnings).subList(1, warnings.length).toArray(
new JSError[warnings.length - 1]);
}
if (warnings.length > 0) {
fail("unexpected warnings(s):\n" + Joiner.on("\n").join(warnings));
}
}
/**
* Parses and type checks the JavaScript code.
*/
private Node parseAndTypeCheck(String js) {
return parseAndTypeCheck(DEFAULT_EXTERNS, js);
}
private Node parseAndTypeCheck(String externs, String js) {
return parseAndTypeCheckWithScope(externs, js).root;
}
/**
* Parses and type checks the JavaScript code and returns the Scope used
* whilst type checking.
*/
private TypeCheckResult parseAndTypeCheckWithScope(String js) {
return parseAndTypeCheckWithScope(DEFAULT_EXTERNS, js);
}
private TypeCheckResult parseAndTypeCheckWithScope(
String externs, String js) {
compiler.init(
Lists.newArrayList(SourceFile.fromCode("[externs]", externs)),
Lists.newArrayList(SourceFile.fromCode("[testcode]", js)),
compiler.getOptions());
Node n = compiler.getInput(new InputId("[testcode]")).getAstRoot(compiler);
Node externsNode = compiler.getInput(new InputId("[externs]"))
.getAstRoot(compiler);
Node externAndJsRoot = new Node(Token.BLOCK, externsNode, n);
externAndJsRoot.setIsSyntheticBlock(true);
assertEquals("parsing error: " +
Joiner.on(", ").join(compiler.getErrors()),
0, compiler.getErrorCount());
Scope s = makeTypeCheck().processForTesting(externsNode, n);
return new TypeCheckResult(n, s);
}
private Node typeCheck(Node n) {
Node externsNode = new Node(Token.BLOCK);
Node externAndJsRoot = new Node(Token.BLOCK, externsNode, n);
externAndJsRoot.setIsSyntheticBlock(true);
makeTypeCheck().processForTesting(null, n);
return n;
}
private TypeCheck makeTypeCheck() {
return new TypeCheck(
compiler,
new SemanticReverseAbstractInterpreter(
compiler.getCodingConvention(), registry),
registry,
reportMissingOverrides,
CheckLevel.OFF);
}
void testTypes(String js, String[] warnings) throws Exception {
Node n = compiler.parseTestCode(js);
assertEquals(0, compiler.getErrorCount());
Node externsNode = new Node(Token.BLOCK);
Node externAndJsRoot = new Node(Token.BLOCK, externsNode, n);
makeTypeCheck().processForTesting(null, n);
assertEquals(0, compiler.getErrorCount());
if (warnings != null) {
assertEquals(warnings.length, compiler.getWarningCount());
JSError[] messages = compiler.getWarnings();
for (int i = 0; i < warnings.length && i < compiler.getWarningCount();
i++) {
assertEquals(warnings[i], messages[i].description);
}
} else {
assertEquals(0, compiler.getWarningCount());
}
}
String suppressMissingProperty(String ... props) {
String result = "function dummy(x) { ";
for (String prop : props) {
result += "x." + prop + " = 3;";
}
return result + "}";
}
private static class TypeCheckResult {
private final Node root;
private final Scope scope;
private TypeCheckResult(Node root, Scope scope) {
this.root = root;
this.scope = scope;
}
}
} | // You are a professional Java test case writer, please create a test case named `testImplementsExtendsLoop` for the issue `Closure-873`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-873
//
// ## Issue-Title:
// Converting from an interface type to a constructor which @implements itself causes stack overflow.
//
// ## Issue-Description:
// // Options: --externs externs/es3.js --property\_renaming OFF --variable\_renaming OFF --jscomp\_warning=checkTypes --js=t.js
//
//
// // File: t.js
// /\*\*
// \* @interface
// \*/
// var OtherType = function() {}
//
// /\*\*
// \* @implements {MyType}
// \* @constructor
// \*/
// var MyType = function() {}
//
// /\*\*
// \* @type {MyType}
// \*/
// var x = /\*\* @type {!OtherType} \*/ (new Object());
//
// Get Infinite recursion in:
//
// PrototypeObjectType.isSubtype @ 350
//
// Options:
//
// - prevent cycles in the inheritance/implements graph
// - detect cycles after they are created and exit compilation before any subsequent passes run
// - detect and remove cycles after they are created but before any subsequent passes run
// - make every subsequent pass robust against cycles in that graph
//
//
public void testImplementsExtendsLoop() throws Exception {
| 9,238 | 4 | 9,230 | test/com/google/javascript/jscomp/TypeCheckTest.java | test | ```markdown
## Issue-ID: Closure-873
## Issue-Title:
Converting from an interface type to a constructor which @implements itself causes stack overflow.
## Issue-Description:
// Options: --externs externs/es3.js --property\_renaming OFF --variable\_renaming OFF --jscomp\_warning=checkTypes --js=t.js
// File: t.js
/\*\*
\* @interface
\*/
var OtherType = function() {}
/\*\*
\* @implements {MyType}
\* @constructor
\*/
var MyType = function() {}
/\*\*
\* @type {MyType}
\*/
var x = /\*\* @type {!OtherType} \*/ (new Object());
Get Infinite recursion in:
PrototypeObjectType.isSubtype @ 350
Options:
- prevent cycles in the inheritance/implements graph
- detect cycles after they are created and exit compilation before any subsequent passes run
- detect and remove cycles after they are created but before any subsequent passes run
- make every subsequent pass robust against cycles in that graph
```
You are a professional Java test case writer, please create a test case named `testImplementsExtendsLoop` for the issue `Closure-873`, utilizing the provided issue report information and the following function signature.
```java
public void testImplementsExtendsLoop() throws Exception {
```
| 9,230 | [
"com.google.javascript.rhino.jstype.NamedType"
] | 9ad5f1236b72367ba5805e9c5389e2c953bcf1f489ed7e12d17e0138fe9e21f8 | public void testImplementsExtendsLoop() throws Exception | // You are a professional Java test case writer, please create a test case named `testImplementsExtendsLoop` for the issue `Closure-873`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-873
//
// ## Issue-Title:
// Converting from an interface type to a constructor which @implements itself causes stack overflow.
//
// ## Issue-Description:
// // Options: --externs externs/es3.js --property\_renaming OFF --variable\_renaming OFF --jscomp\_warning=checkTypes --js=t.js
//
//
// // File: t.js
// /\*\*
// \* @interface
// \*/
// var OtherType = function() {}
//
// /\*\*
// \* @implements {MyType}
// \* @constructor
// \*/
// var MyType = function() {}
//
// /\*\*
// \* @type {MyType}
// \*/
// var x = /\*\* @type {!OtherType} \*/ (new Object());
//
// Get Infinite recursion in:
//
// PrototypeObjectType.isSubtype @ 350
//
// Options:
//
// - prevent cycles in the inheritance/implements graph
// - detect cycles after they are created and exit compilation before any subsequent passes run
// - detect and remove cycles after they are created but before any subsequent passes run
// - make every subsequent pass robust against cycles in that graph
//
//
| Closure | /*
* Copyright 2006 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import com.google.common.base.Joiner;
import com.google.common.collect.Lists;
import com.google.common.collect.Sets;
import com.google.javascript.jscomp.Scope.Var;
import com.google.javascript.jscomp.type.ClosureReverseAbstractInterpreter;
import com.google.javascript.jscomp.type.SemanticReverseAbstractInterpreter;
import com.google.javascript.rhino.InputId;
import com.google.javascript.rhino.Node;
import com.google.javascript.rhino.Token;
import com.google.javascript.rhino.jstype.FunctionType;
import com.google.javascript.rhino.jstype.JSType;
import com.google.javascript.rhino.jstype.JSTypeNative;
import com.google.javascript.rhino.jstype.ObjectType;
import com.google.javascript.rhino.testing.Asserts;
import java.util.Arrays;
import java.util.List;
import java.util.Set;
/**
* Tests {@link TypeCheck}.
*
*/
public class TypeCheckTest extends CompilerTypeTestCase {
private CheckLevel reportMissingOverrides = CheckLevel.WARNING;
@Override
public void setUp() throws Exception {
super.setUp();
reportMissingOverrides = CheckLevel.WARNING;
}
public void testInitialTypingScope() {
Scope s = new TypedScopeCreator(compiler,
CodingConventions.getDefault()).createInitialScope(
new Node(Token.BLOCK));
assertTypeEquals(ARRAY_FUNCTION_TYPE, s.getVar("Array").getType());
assertTypeEquals(BOOLEAN_OBJECT_FUNCTION_TYPE,
s.getVar("Boolean").getType());
assertTypeEquals(DATE_FUNCTION_TYPE, s.getVar("Date").getType());
assertTypeEquals(ERROR_FUNCTION_TYPE, s.getVar("Error").getType());
assertTypeEquals(EVAL_ERROR_FUNCTION_TYPE,
s.getVar("EvalError").getType());
assertTypeEquals(NUMBER_OBJECT_FUNCTION_TYPE,
s.getVar("Number").getType());
assertTypeEquals(OBJECT_FUNCTION_TYPE, s.getVar("Object").getType());
assertTypeEquals(RANGE_ERROR_FUNCTION_TYPE,
s.getVar("RangeError").getType());
assertTypeEquals(REFERENCE_ERROR_FUNCTION_TYPE,
s.getVar("ReferenceError").getType());
assertTypeEquals(REGEXP_FUNCTION_TYPE, s.getVar("RegExp").getType());
assertTypeEquals(STRING_OBJECT_FUNCTION_TYPE,
s.getVar("String").getType());
assertTypeEquals(SYNTAX_ERROR_FUNCTION_TYPE,
s.getVar("SyntaxError").getType());
assertTypeEquals(TYPE_ERROR_FUNCTION_TYPE,
s.getVar("TypeError").getType());
assertTypeEquals(URI_ERROR_FUNCTION_TYPE,
s.getVar("URIError").getType());
}
public void testPrivateType() throws Exception {
testTypes(
"/** @private {number} */ var x = false;",
"initializing variable\n" +
"found : boolean\n" +
"required: number");
}
public void testTypeCheck1() throws Exception {
testTypes("/**@return {void}*/function foo(){ if (foo()) return; }");
}
public void testTypeCheck2() throws Exception {
testTypes("/**@return {void}*/function foo(){ var x=foo(); x--; }",
"increment/decrement\n" +
"found : undefined\n" +
"required: number");
}
public void testTypeCheck4() throws Exception {
testTypes("/**@return {void}*/function foo(){ !foo(); }");
}
public void testTypeCheck5() throws Exception {
testTypes("/**@return {void}*/function foo(){ var a = +foo(); }",
"sign operator\n" +
"found : undefined\n" +
"required: number");
}
public void testTypeCheck6() throws Exception {
testTypes(
"/**@return {void}*/function foo(){" +
"/** @type {undefined|number} */var a;if (a == foo())return;}");
}
public void testTypeCheck8() throws Exception {
testTypes("/**@return {void}*/function foo(){do {} while (foo());}");
}
public void testTypeCheck9() throws Exception {
testTypes("/**@return {void}*/function foo(){while (foo());}");
}
public void testTypeCheck10() throws Exception {
testTypes("/**@return {void}*/function foo(){for (;foo(););}");
}
public void testTypeCheck11() throws Exception {
testTypes("/**@type !Number */var a;" +
"/**@type !String */var b;" +
"a = b;",
"assignment\n" +
"found : String\n" +
"required: Number");
}
public void testTypeCheck12() throws Exception {
testTypes("/**@return {!Object}*/function foo(){var a = 3^foo();}",
"bad right operand to bitwise operator\n" +
"found : Object\n" +
"required: (boolean|null|number|string|undefined)");
}
public void testTypeCheck13() throws Exception {
testTypes("/**@type {!Number|!String}*/var i; i=/xx/;",
"assignment\n" +
"found : RegExp\n" +
"required: (Number|String)");
}
public void testTypeCheck14() throws Exception {
testTypes("/**@param opt_a*/function foo(opt_a){}");
}
public void testTypeCheck15() throws Exception {
testTypes("/**@type {Number|null} */var x;x=null;x=10;",
"assignment\n" +
"found : number\n" +
"required: (Number|null)");
}
public void testTypeCheck16() throws Exception {
testTypes("/**@type {Number|null} */var x='';",
"initializing variable\n" +
"found : string\n" +
"required: (Number|null)");
}
public void testTypeCheck17() throws Exception {
testTypes("/**@return {Number}\n@param {Number} opt_foo */\n" +
"function a(opt_foo){\nreturn /**@type {Number}*/(opt_foo);\n}");
}
public void testTypeCheck18() throws Exception {
testTypes("/**@return {RegExp}\n*/\n function a(){return new RegExp();}");
}
public void testTypeCheck19() throws Exception {
testTypes("/**@return {Array}\n*/\n function a(){return new Array();}");
}
public void testTypeCheck20() throws Exception {
testTypes("/**@return {Date}\n*/\n function a(){return new Date();}");
}
public void testTypeCheckBasicDowncast() throws Exception {
testTypes("/** @constructor */function foo() {}\n" +
"/** @type {Object} */ var bar = new foo();\n");
}
public void testTypeCheckNoDowncastToNumber() throws Exception {
testTypes("/** @constructor */function foo() {}\n" +
"/** @type {!Number} */ var bar = new foo();\n",
"initializing variable\n" +
"found : foo\n" +
"required: Number");
}
public void testTypeCheck21() throws Exception {
testTypes("/** @type Array.<String> */var foo;");
}
public void testTypeCheck22() throws Exception {
testTypes("/** @param {Element|Object} p */\nfunction foo(p){}\n" +
"/** @constructor */function Element(){}\n" +
"/** @type {Element|Object} */var v;\n" +
"foo(v);\n");
}
public void testTypeCheck23() throws Exception {
testTypes("/** @type {(Object,Null)} */var foo; foo = null;");
}
public void testTypeCheck24() throws Exception {
testTypes("/** @constructor */function MyType(){}\n" +
"/** @type {(MyType,Null)} */var foo; foo = null;");
}
public void testTypeCheckDefaultExterns() throws Exception {
testTypes("/** @param {string} x */ function f(x) {}" +
"f([].length);" ,
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testTypeCheckCustomExterns() throws Exception {
testTypes(
DEFAULT_EXTERNS + "/** @type {boolean} */ Array.prototype.oogabooga;",
"/** @param {string} x */ function f(x) {}" +
"f([].oogabooga);" ,
"actual parameter 1 of f does not match formal parameter\n" +
"found : boolean\n" +
"required: string", false);
}
public void testTypeCheckCustomExterns2() throws Exception {
testTypes(
DEFAULT_EXTERNS + "/** @enum {string} */ var Enum = {FOO: 1, BAR: 1};",
"/** @param {Enum} x */ function f(x) {} f(Enum.FOO); f(true);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : boolean\n" +
"required: Enum.<string>",
false);
}
public void testParameterizedArray1() throws Exception {
testTypes("/** @param {!Array.<number>} a\n" +
"* @return {string}\n" +
"*/ var f = function(a) { return a[0]; };",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testParameterizedArray2() throws Exception {
testTypes("/** @param {!Array.<!Array.<number>>} a\n" +
"* @return {number}\n" +
"*/ var f = function(a) { return a[0]; };",
"inconsistent return type\n" +
"found : Array.<number>\n" +
"required: number");
}
public void testParameterizedArray3() throws Exception {
testTypes("/** @param {!Array.<number>} a\n" +
"* @return {number}\n" +
"*/ var f = function(a) { a[1] = 0; return a[0]; };");
}
public void testParameterizedArray4() throws Exception {
testTypes("/** @param {!Array.<number>} a\n" +
"*/ var f = function(a) { a[0] = 'a'; };",
"assignment\n" +
"found : string\n" +
"required: number");
}
public void testParameterizedArray5() throws Exception {
testTypes("/** @param {!Array.<*>} a\n" +
"*/ var f = function(a) { a[0] = 'a'; };");
}
public void testParameterizedArray6() throws Exception {
testTypes("/** @param {!Array.<*>} a\n" +
"* @return {string}\n" +
"*/ var f = function(a) { return a[0]; };",
"inconsistent return type\n" +
"found : *\n" +
"required: string");
}
public void testParameterizedArray7() throws Exception {
testTypes("/** @param {?Array.<number>} a\n" +
"* @return {string}\n" +
"*/ var f = function(a) { return a[0]; };",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testParameterizedObject1() throws Exception {
testTypes("/** @param {!Object.<number>} a\n" +
"* @return {string}\n" +
"*/ var f = function(a) { return a[0]; };",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testParameterizedObject2() throws Exception {
testTypes("/** @param {!Object.<string,number>} a\n" +
"* @return {string}\n" +
"*/ var f = function(a) { return a['x']; };",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testParameterizedObject3() throws Exception {
testTypes("/** @param {!Object.<number,string>} a\n" +
"* @return {string}\n" +
"*/ var f = function(a) { return a['x']; };",
"restricted index type\n" +
"found : string\n" +
"required: number");
}
public void testParameterizedObject4() throws Exception {
testTypes("/** @enum {string} */ var E = {A: 'a', B: 'b'};\n" +
"/** @param {!Object.<E,string>} a\n" +
"* @return {string}\n" +
"*/ var f = function(a) { return a['x']; };",
"restricted index type\n" +
"found : string\n" +
"required: E.<string>");
}
public void testParameterizedObject5() throws Exception {
testTypes("/** @constructor */ function F() {" +
" /** @type {Object.<number, string>} */ this.numbers = {};" +
"}" +
"(new F()).numbers['ten'] = '10';",
"restricted index type\n" +
"found : string\n" +
"required: number");
}
public void testUnionOfFunctionAndType() throws Exception {
testTypes("/** @type {null|(function(Number):void)} */ var a;" +
"/** @type {(function(Number):void)|null} */ var b = null; a = b;");
}
public void testOptionalParameterComparedToUndefined() throws Exception {
testTypes("/**@param opt_a {Number}*/function foo(opt_a)" +
"{if (opt_a==undefined) var b = 3;}");
}
public void testOptionalAllType() throws Exception {
testTypes("/** @param {*} opt_x */function f(opt_x) { return opt_x }\n" +
"/** @type {*} */var y;\n" +
"f(y);");
}
public void testOptionalUnknownNamedType() throws Exception {
testTypes("/** @param {!T} opt_x\n@return {undefined} */\n" +
"function f(opt_x) { return opt_x; }\n" +
"/** @constructor */var T = function() {};",
"inconsistent return type\n" +
"found : (T|undefined)\n" +
"required: undefined");
}
public void testOptionalArgFunctionParam() throws Exception {
testTypes("/** @param {function(number=)} a */" +
"function f(a) {a()};");
}
public void testOptionalArgFunctionParam2() throws Exception {
testTypes("/** @param {function(number=)} a */" +
"function f(a) {a(3)};");
}
public void testOptionalArgFunctionParam3() throws Exception {
testTypes("/** @param {function(number=)} a */" +
"function f(a) {a(undefined)};");
}
public void testOptionalArgFunctionParam4() throws Exception {
String expectedWarning = "Function a: called with 2 argument(s). " +
"Function requires at least 0 argument(s) and no more than 1 " +
"argument(s).";
testTypes("/** @param {function(number=)} a */function f(a) {a(3,4)};",
expectedWarning, false);
}
public void testOptionalArgFunctionParamError() throws Exception {
String expectedWarning =
"Bad type annotation. variable length argument must be last";
testTypes("/** @param {function(...[number], number=)} a */" +
"function f(a) {};", expectedWarning, false);
}
public void testOptionalNullableArgFunctionParam() throws Exception {
testTypes("/** @param {function(?number=)} a */" +
"function f(a) {a()};");
}
public void testOptionalNullableArgFunctionParam2() throws Exception {
testTypes("/** @param {function(?number=)} a */" +
"function f(a) {a(null)};");
}
public void testOptionalNullableArgFunctionParam3() throws Exception {
testTypes("/** @param {function(?number=)} a */" +
"function f(a) {a(3)};");
}
public void testOptionalArgFunctionReturn() throws Exception {
testTypes("/** @return {function(number=)} */" +
"function f() { return function(opt_x) { }; };" +
"f()()");
}
public void testOptionalArgFunctionReturn2() throws Exception {
testTypes("/** @return {function(Object=)} */" +
"function f() { return function(opt_x) { }; };" +
"f()({})");
}
public void testBooleanType() throws Exception {
testTypes("/**@type {boolean} */var x = 1 < 2;");
}
public void testBooleanReduction1() throws Exception {
testTypes("/**@type {string} */var x; x = null || \"a\";");
}
public void testBooleanReduction2() throws Exception {
// It's important for the type system to recognize that in no case
// can the boolean expression evaluate to a boolean value.
testTypes("/** @param {string} s\n @return {string} */" +
"(function(s) { return ((s == 'a') && s) || 'b'; })");
}
public void testBooleanReduction3() throws Exception {
testTypes("/** @param {string} s\n @return {string?} */" +
"(function(s) { return s && null && 3; })");
}
public void testBooleanReduction4() throws Exception {
testTypes("/** @param {Object} x\n @return {Object} */" +
"(function(x) { return null || x || null ; })");
}
public void testBooleanReduction5() throws Exception {
testTypes("/**\n" +
"* @param {Array|string} x\n" +
"* @return {string?}\n" +
"*/\n" +
"var f = function(x) {\n" +
"if (!x || typeof x == 'string') {\n" +
"return x;\n" +
"}\n" +
"return null;\n" +
"};");
}
public void testBooleanReduction6() throws Exception {
testTypes("/**\n" +
"* @param {Array|string|null} x\n" +
"* @return {string?}\n" +
"*/\n" +
"var f = function(x) {\n" +
"if (!(x && typeof x != 'string')) {\n" +
"return x;\n" +
"}\n" +
"return null;\n" +
"};");
}
public void testBooleanReduction7() throws Exception {
testTypes("/** @constructor */var T = function() {};\n" +
"/**\n" +
"* @param {Array|T} x\n" +
"* @return {null}\n" +
"*/\n" +
"var f = function(x) {\n" +
"if (!x) {\n" +
"return x;\n" +
"}\n" +
"return null;\n" +
"};");
}
public void testNullAnd() throws Exception {
testTypes("/** @type null */var x;\n" +
"/** @type number */var r = x && x;",
"initializing variable\n" +
"found : null\n" +
"required: number");
}
public void testNullOr() throws Exception {
testTypes("/** @type null */var x;\n" +
"/** @type number */var r = x || x;",
"initializing variable\n" +
"found : null\n" +
"required: number");
}
public void testBooleanPreservation1() throws Exception {
testTypes("/**@type {string} */var x = \"a\";" +
"x = ((x == \"a\") && x) || x == \"b\";",
"assignment\n" +
"found : (boolean|string)\n" +
"required: string");
}
public void testBooleanPreservation2() throws Exception {
testTypes("/**@type {string} */var x = \"a\"; x = (x == \"a\") || x;",
"assignment\n" +
"found : (boolean|string)\n" +
"required: string");
}
public void testBooleanPreservation3() throws Exception {
testTypes("/** @param {Function?} x\n @return {boolean?} */" +
"function f(x) { return x && x == \"a\"; }",
"condition always evaluates to false\n" +
"left : Function\n" +
"right: string");
}
public void testBooleanPreservation4() throws Exception {
testTypes("/** @param {Function?|boolean} x\n @return {boolean} */" +
"function f(x) { return x && x == \"a\"; }",
"inconsistent return type\n" +
"found : (boolean|null)\n" +
"required: boolean");
}
public void testTypeOfReduction1() throws Exception {
testTypes("/** @param {string|number} x\n @return {string} */ " +
"function f(x) { return typeof x == 'number' ? String(x) : x; }");
}
public void testTypeOfReduction2() throws Exception {
testTypes("/** @param {string|number} x\n @return {string} */ " +
"function f(x) { return typeof x != 'string' ? String(x) : x; }");
}
public void testTypeOfReduction3() throws Exception {
testTypes("/** @param {number|null} x\n @return {number} */ " +
"function f(x) { return typeof x == 'object' ? 1 : x; }");
}
public void testTypeOfReduction4() throws Exception {
testTypes("/** @param {Object|undefined} x\n @return {Object} */ " +
"function f(x) { return typeof x == 'undefined' ? {} : x; }");
}
public void testTypeOfReduction5() throws Exception {
testTypes("/** @enum {string} */ var E = {A: 'a', B: 'b'};\n" +
"/** @param {!E|number} x\n @return {string} */ " +
"function f(x) { return typeof x != 'number' ? x : 'a'; }");
}
public void testTypeOfReduction6() throws Exception {
testTypes("/** @param {number|string} x\n@return {string} */\n" +
"function f(x) {\n" +
"return typeof x == 'string' && x.length == 3 ? x : 'a';\n" +
"}");
}
public void testTypeOfReduction7() throws Exception {
testTypes("/** @return {string} */var f = function(x) { " +
"return typeof x == 'number' ? x : 'a'; }",
"inconsistent return type\n" +
"found : (number|string)\n" +
"required: string");
}
public void testTypeOfReduction8() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @param {number|string} x\n@return {string} */\n" +
"function f(x) {\n" +
"return goog.isString(x) && x.length == 3 ? x : 'a';\n" +
"}", null);
}
public void testTypeOfReduction9() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @param {!Array|string} x\n@return {string} */\n" +
"function f(x) {\n" +
"return goog.isArray(x) ? 'a' : x;\n" +
"}", null);
}
public void testTypeOfReduction10() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @param {Array|string} x\n@return {Array} */\n" +
"function f(x) {\n" +
"return goog.isArray(x) ? x : [];\n" +
"}", null);
}
public void testTypeOfReduction11() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @param {Array|string} x\n@return {Array} */\n" +
"function f(x) {\n" +
"return goog.isObject(x) ? x : [];\n" +
"}", null);
}
public void testTypeOfReduction12() throws Exception {
testTypes("/** @enum {string} */ var E = {A: 'a', B: 'b'};\n" +
"/** @param {E|Array} x\n @return {Array} */ " +
"function f(x) { return typeof x == 'object' ? x : []; }");
}
public void testTypeOfReduction13() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @enum {string} */ var E = {A: 'a', B: 'b'};\n" +
"/** @param {E|Array} x\n@return {Array} */ " +
"function f(x) { return goog.isObject(x) ? x : []; }", null);
}
public void testTypeOfReduction14() throws Exception {
// Don't do type inference on GETELEMs.
testClosureTypes(
CLOSURE_DEFS +
"function f(x) { " +
" return goog.isString(arguments[0]) ? arguments[0] : 0;" +
"}", null);
}
public void testTypeOfReduction15() throws Exception {
// Don't do type inference on GETELEMs.
testClosureTypes(
CLOSURE_DEFS +
"function f(x) { " +
" return typeof arguments[0] == 'string' ? arguments[0] : 0;" +
"}", null);
}
public void testTypeOfReduction16() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @interface */ function I() {}\n" +
"/**\n" +
" * @param {*} x\n" +
" * @return {I}\n" +
" */\n" +
"function f(x) { " +
" if(goog.isObject(x)) {" +
" return /** @type {I} */(x);" +
" }" +
" return null;" +
"}", null);
}
public void testQualifiedNameReduction1() throws Exception {
testTypes("var x = {}; /** @type {string?} */ x.a = 'a';\n" +
"/** @return {string} */ var f = function() {\n" +
"return x.a ? x.a : 'a'; }");
}
public void testQualifiedNameReduction2() throws Exception {
testTypes("/** @param {string?} a\n@constructor */ var T = " +
"function(a) {this.a = a};\n" +
"/** @return {string} */ T.prototype.f = function() {\n" +
"return this.a ? this.a : 'a'; }");
}
public void testQualifiedNameReduction3() throws Exception {
testTypes("/** @param {string|Array} a\n@constructor */ var T = " +
"function(a) {this.a = a};\n" +
"/** @return {string} */ T.prototype.f = function() {\n" +
"return typeof this.a == 'string' ? this.a : 'a'; }");
}
public void testQualifiedNameReduction4() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @param {string|Array} a\n@constructor */ var T = " +
"function(a) {this.a = a};\n" +
"/** @return {string} */ T.prototype.f = function() {\n" +
"return goog.isString(this.a) ? this.a : 'a'; }", null);
}
public void testQualifiedNameReduction5a() throws Exception {
testTypes("var x = {/** @type {string} */ a:'b' };\n" +
"/** @return {string} */ var f = function() {\n" +
"return x.a; }");
}
public void testQualifiedNameReduction5b() throws Exception {
testTypes(
"var x = {/** @type {number} */ a:12 };\n" +
"/** @return {string} */\n" +
"var f = function() {\n" +
" return x.a;\n" +
"}",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testQualifiedNameReduction5c() throws Exception {
testTypes(
"/** @return {string} */ var f = function() {\n" +
"var x = {/** @type {number} */ a:0 };\n" +
"return (x.a) ? (x.a) : 'a'; }",
"inconsistent return type\n" +
"found : (number|string)\n" +
"required: string");
}
public void testQualifiedNameReduction6() throws Exception {
testTypes(
"/** @return {string} */ var f = function() {\n" +
"var x = {/** @return {string?} */ get a() {return 'a'}};\n" +
"return x.a ? x.a : 'a'; }");
}
public void testQualifiedNameReduction7() throws Exception {
testTypes(
"/** @return {string} */ var f = function() {\n" +
"var x = {/** @return {number} */ get a() {return 12}};\n" +
"return x.a; }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testQualifiedNameReduction7a() throws Exception {
// It would be nice to find a way to make this an error.
testTypes(
"/** @return {string} */ var f = function() {\n" +
"var x = {get a() {return 12}};\n" +
"return x.a; }");
}
public void testQualifiedNameReduction8() throws Exception {
testTypes(
"/** @return {string} */ var f = function() {\n" +
"var x = {get a() {return 'a'}};\n" +
"return x.a ? x.a : 'a'; }");
}
public void testQualifiedNameReduction9() throws Exception {
testTypes(
"/** @return {string} */ var f = function() {\n" +
"var x = { /** @param {string} b */ set a(b) {}};\n" +
"return x.a ? x.a : 'a'; }");
}
public void testQualifiedNameReduction10() throws Exception {
// TODO(johnlenz): separate setter property types from getter property
// types.
testTypes(
"/** @return {string} */ var f = function() {\n" +
"var x = { /** @param {number} b */ set a(b) {}};\n" +
"return x.a ? x.a : 'a'; }",
"inconsistent return type\n" +
"found : (number|string)\n" +
"required: string");
}
public void testObjLitDef1a() throws Exception {
testTypes(
"var x = {/** @type {number} */ a:12 };\n" +
"x.a = 'a';",
"assignment to property a of x\n" +
"found : string\n" +
"required: number");
}
public void testObjLitDef1b() throws Exception {
testTypes(
"function f(){" +
"var x = {/** @type {number} */ a:12 };\n" +
"x.a = 'a';" +
"};\n" +
"f();",
"assignment to property a of x\n" +
"found : string\n" +
"required: number");
}
public void testObjLitDef2a() throws Exception {
testTypes(
"var x = {/** @param {number} b */ set a(b){} };\n" +
"x.a = 'a';",
"assignment to property a of x\n" +
"found : string\n" +
"required: number");
}
public void testObjLitDef2b() throws Exception {
testTypes(
"function f(){" +
"var x = {/** @param {number} b */ set a(b){} };\n" +
"x.a = 'a';" +
"};\n" +
"f();",
"assignment to property a of x\n" +
"found : string\n" +
"required: number");
}
public void testObjLitDef3a() throws Exception {
testTypes(
"/** @type {string} */ var y;\n" +
"var x = {/** @return {number} */ get a(){} };\n" +
"y = x.a;",
"assignment\n" +
"found : number\n" +
"required: string");
}
public void testObjLitDef3b() throws Exception {
testTypes(
"/** @type {string} */ var y;\n" +
"function f(){" +
"var x = {/** @return {number} */ get a(){} };\n" +
"y = x.a;" +
"};\n" +
"f();",
"assignment\n" +
"found : number\n" +
"required: string");
}
public void testObjLitDef4() throws Exception {
testTypes(
"var x = {" +
"/** @return {number} */ a:12 };\n",
"assignment to property a of {a: function (): number}\n" +
"found : number\n" +
"required: function (): number");
}
public void testObjLitDef5() throws Exception {
testTypes(
"var x = {};\n" +
"/** @return {number} */ x.a = 12;\n",
"assignment to property a of x\n" +
"found : number\n" +
"required: function (): number");
}
public void testInstanceOfReduction1() throws Exception {
testTypes("/** @constructor */ var T = function() {};\n" +
"/** @param {T|string} x\n@return {T} */\n" +
"var f = function(x) {\n" +
"if (x instanceof T) { return x; } else { return new T(); }\n" +
"};");
}
public void testInstanceOfReduction2() throws Exception {
testTypes("/** @constructor */ var T = function() {};\n" +
"/** @param {!T|string} x\n@return {string} */\n" +
"var f = function(x) {\n" +
"if (x instanceof T) { return ''; } else { return x; }\n" +
"};");
}
public void testUndeclaredGlobalProperty1() throws Exception {
testTypes("/** @const */ var x = {}; x.y = null;" +
"function f(a) { x.y = a; }" +
"/** @param {string} a */ function g(a) { }" +
"function h() { g(x.y); }");
}
public void testUndeclaredGlobalProperty2() throws Exception {
testTypes("/** @const */ var x = {}; x.y = null;" +
"function f() { x.y = 3; }" +
"/** @param {string} a */ function g(a) { }" +
"function h() { g(x.y); }",
"actual parameter 1 of g does not match formal parameter\n" +
"found : (null|number)\n" +
"required: string");
}
public void testLocallyInferredGlobalProperty1() throws Exception {
// We used to have a bug where x.y.z leaked from f into h.
testTypes(
"/** @constructor */ function F() {}" +
"/** @type {number} */ F.prototype.z;" +
"/** @const */ var x = {}; /** @type {F} */ x.y;" +
"function f() { x.y.z = 'abc'; }" +
"/** @param {number} x */ function g(x) {}" +
"function h() { g(x.y.z); }",
"assignment to property z of F\n" +
"found : string\n" +
"required: number");
}
public void testPropertyInferredPropagation() throws Exception {
testTypes("/** @return {Object} */function f() { return {}; }\n" +
"function g() { var x = f(); if (x.p) x.a = 'a'; else x.a = 'b'; }\n" +
"function h() { var x = f(); x.a = false; }");
}
public void testPropertyInference1() throws Exception {
testTypes(
"/** @constructor */ function F() { this.x_ = true; }" +
"/** @return {string} */" +
"F.prototype.bar = function() { if (this.x_) return this.x_; };",
"inconsistent return type\n" +
"found : boolean\n" +
"required: string");
}
public void testPropertyInference2() throws Exception {
testTypes(
"/** @constructor */ function F() { this.x_ = true; }" +
"F.prototype.baz = function() { this.x_ = null; };" +
"/** @return {string} */" +
"F.prototype.bar = function() { if (this.x_) return this.x_; };",
"inconsistent return type\n" +
"found : boolean\n" +
"required: string");
}
public void testPropertyInference3() throws Exception {
testTypes(
"/** @constructor */ function F() { this.x_ = true; }" +
"F.prototype.baz = function() { this.x_ = 3; };" +
"/** @return {string} */" +
"F.prototype.bar = function() { if (this.x_) return this.x_; };",
"inconsistent return type\n" +
"found : (boolean|number)\n" +
"required: string");
}
public void testPropertyInference4() throws Exception {
testTypes(
"/** @constructor */ function F() { }" +
"F.prototype.x_ = 3;" +
"/** @return {string} */" +
"F.prototype.bar = function() { if (this.x_) return this.x_; };",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testPropertyInference5() throws Exception {
testTypes(
"/** @constructor */ function F() { }" +
"F.prototype.baz = function() { this.x_ = 3; };" +
"/** @return {string} */" +
"F.prototype.bar = function() { if (this.x_) return this.x_; };");
}
public void testPropertyInference6() throws Exception {
testTypes(
"/** @constructor */ function F() { }" +
"(new F).x_ = 3;" +
"/** @return {string} */" +
"F.prototype.bar = function() { return this.x_; };");
}
public void testPropertyInference7() throws Exception {
testTypes(
"/** @constructor */ function F() { this.x_ = true; }" +
"(new F).x_ = 3;" +
"/** @return {string} */" +
"F.prototype.bar = function() { return this.x_; };",
"inconsistent return type\n" +
"found : boolean\n" +
"required: string");
}
public void testPropertyInference8() throws Exception {
testTypes(
"/** @constructor */ function F() { " +
" /** @type {string} */ this.x_ = 'x';" +
"}" +
"(new F).x_ = 3;" +
"/** @return {string} */" +
"F.prototype.bar = function() { return this.x_; };",
"assignment to property x_ of F\n" +
"found : number\n" +
"required: string");
}
public void testPropertyInference9() throws Exception {
testTypes(
"/** @constructor */ function A() {}" +
"/** @return {function(): ?} */ function f() { " +
" return function() {};" +
"}" +
"var g = f();" +
"/** @type {number} */ g.prototype.bar_ = null;",
"assignment\n" +
"found : null\n" +
"required: number");
}
public void testPropertyInference10() throws Exception {
// NOTE(nicksantos): There used to be a bug where a property
// on the prototype of one structural function would leak onto
// the prototype of other variables with the same structural
// function type.
testTypes(
"/** @constructor */ function A() {}" +
"/** @return {function(): ?} */ function f() { " +
" return function() {};" +
"}" +
"var g = f();" +
"/** @type {number} */ g.prototype.bar_ = 1;" +
"var h = f();" +
"/** @type {string} */ h.prototype.bar_ = 1;",
"assignment\n" +
"found : number\n" +
"required: string");
}
public void testNoPersistentTypeInferenceForObjectProperties()
throws Exception {
testTypes("/** @param {Object} o\n@param {string} x */\n" +
"function s1(o,x) { o.x = x; }\n" +
"/** @param {Object} o\n@return {string} */\n" +
"function g1(o) { return typeof o.x == 'undefined' ? '' : o.x; }\n" +
"/** @param {Object} o\n@param {number} x */\n" +
"function s2(o,x) { o.x = x; }\n" +
"/** @param {Object} o\n@return {number} */\n" +
"function g2(o) { return typeof o.x == 'undefined' ? 0 : o.x; }");
}
public void testNoPersistentTypeInferenceForFunctionProperties()
throws Exception {
testTypes("/** @param {Function} o\n@param {string} x */\n" +
"function s1(o,x) { o.x = x; }\n" +
"/** @param {Function} o\n@return {string} */\n" +
"function g1(o) { return typeof o.x == 'undefined' ? '' : o.x; }\n" +
"/** @param {Function} o\n@param {number} x */\n" +
"function s2(o,x) { o.x = x; }\n" +
"/** @param {Function} o\n@return {number} */\n" +
"function g2(o) { return typeof o.x == 'undefined' ? 0 : o.x; }");
}
public void testObjectPropertyTypeInferredInLocalScope1() throws Exception {
testTypes("/** @param {!Object} o\n@return {string} */\n" +
"function f(o) { o.x = 1; return o.x; }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testObjectPropertyTypeInferredInLocalScope2() throws Exception {
testTypes("/**@param {!Object} o\n@param {number?} x\n@return {string}*/" +
"function f(o, x) { o.x = 'a';\nif (x) {o.x = x;}\nreturn o.x; }",
"inconsistent return type\n" +
"found : (number|string)\n" +
"required: string");
}
public void testObjectPropertyTypeInferredInLocalScope3() throws Exception {
testTypes("/**@param {!Object} o\n@param {number?} x\n@return {string}*/" +
"function f(o, x) { if (x) {o.x = x;} else {o.x = 'a';}\nreturn o.x; }",
"inconsistent return type\n" +
"found : (number|string)\n" +
"required: string");
}
public void testMismatchingOverridingInferredPropertyBeforeDeclaredProperty1()
throws Exception {
testTypes("/** @constructor */var T = function() { this.x = ''; };\n" +
"/** @type {number} */ T.prototype.x = 0;",
"assignment to property x of T\n" +
"found : string\n" +
"required: number");
}
public void testMismatchingOverridingInferredPropertyBeforeDeclaredProperty2()
throws Exception {
testTypes("/** @constructor */var T = function() { this.x = ''; };\n" +
"/** @type {number} */ T.prototype.x;",
"assignment to property x of T\n" +
"found : string\n" +
"required: number");
}
public void testMismatchingOverridingInferredPropertyBeforeDeclaredProperty3()
throws Exception {
testTypes("/** @type {Object} */ var n = {};\n" +
"/** @constructor */ n.T = function() { this.x = ''; };\n" +
"/** @type {number} */ n.T.prototype.x = 0;",
"assignment to property x of n.T\n" +
"found : string\n" +
"required: number");
}
public void testMismatchingOverridingInferredPropertyBeforeDeclaredProperty4()
throws Exception {
testTypes("var n = {};\n" +
"/** @constructor */ n.T = function() { this.x = ''; };\n" +
"/** @type {number} */ n.T.prototype.x = 0;",
"assignment to property x of n.T\n" +
"found : string\n" +
"required: number");
}
public void testPropertyUsedBeforeDefinition1() throws Exception {
testTypes("/** @constructor */ var T = function() {};\n" +
"/** @return {string} */" +
"T.prototype.f = function() { return this.g(); };\n" +
"/** @return {number} */ T.prototype.g = function() { return 1; };\n",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testPropertyUsedBeforeDefinition2() throws Exception {
testTypes("var n = {};\n" +
"/** @constructor */ n.T = function() {};\n" +
"/** @return {string} */" +
"n.T.prototype.f = function() { return this.g(); };\n" +
"/** @return {number} */ n.T.prototype.g = function() { return 1; };\n",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testAdd1() throws Exception {
testTypes("/**@return {void}*/function foo(){var a = 'abc'+foo();}");
}
public void testAdd2() throws Exception {
testTypes("/**@return {void}*/function foo(){var a = foo()+4;}");
}
public void testAdd3() throws Exception {
testTypes("/** @type {string} */ var a = 'a';" +
"/** @type {string} */ var b = 'b';" +
"/** @type {string} */ var c = a + b;");
}
public void testAdd4() throws Exception {
testTypes("/** @type {number} */ var a = 5;" +
"/** @type {string} */ var b = 'b';" +
"/** @type {string} */ var c = a + b;");
}
public void testAdd5() throws Exception {
testTypes("/** @type {string} */ var a = 'a';" +
"/** @type {number} */ var b = 5;" +
"/** @type {string} */ var c = a + b;");
}
public void testAdd6() throws Exception {
testTypes("/** @type {number} */ var a = 5;" +
"/** @type {number} */ var b = 5;" +
"/** @type {number} */ var c = a + b;");
}
public void testAdd7() throws Exception {
testTypes("/** @type {number} */ var a = 5;" +
"/** @type {string} */ var b = 'b';" +
"/** @type {number} */ var c = a + b;",
"initializing variable\n" +
"found : string\n" +
"required: number");
}
public void testAdd8() throws Exception {
testTypes("/** @type {string} */ var a = 'a';" +
"/** @type {number} */ var b = 5;" +
"/** @type {number} */ var c = a + b;",
"initializing variable\n" +
"found : string\n" +
"required: number");
}
public void testAdd9() throws Exception {
testTypes("/** @type {number} */ var a = 5;" +
"/** @type {number} */ var b = 5;" +
"/** @type {string} */ var c = a + b;",
"initializing variable\n" +
"found : number\n" +
"required: string");
}
public void testAdd10() throws Exception {
// d.e.f will have unknown type.
testTypes(
suppressMissingProperty("e", "f") +
"/** @type {number} */ var a = 5;" +
"/** @type {string} */ var c = a + d.e.f;");
}
public void testAdd11() throws Exception {
// d.e.f will have unknown type.
testTypes(
suppressMissingProperty("e", "f") +
"/** @type {number} */ var a = 5;" +
"/** @type {number} */ var c = a + d.e.f;");
}
public void testAdd12() throws Exception {
testTypes("/** @return {(number,string)} */ function a() { return 5; }" +
"/** @type {number} */ var b = 5;" +
"/** @type {boolean} */ var c = a() + b;",
"initializing variable\n" +
"found : (number|string)\n" +
"required: boolean");
}
public void testAdd13() throws Exception {
testTypes("/** @type {number} */ var a = 5;" +
"/** @return {(number,string)} */ function b() { return 5; }" +
"/** @type {boolean} */ var c = a + b();",
"initializing variable\n" +
"found : (number|string)\n" +
"required: boolean");
}
public void testAdd14() throws Exception {
testTypes("/** @type {(null,string)} */ var a = null;" +
"/** @type {number} */ var b = 5;" +
"/** @type {boolean} */ var c = a + b;",
"initializing variable\n" +
"found : (number|string)\n" +
"required: boolean");
}
public void testAdd15() throws Exception {
testTypes("/** @type {number} */ var a = 5;" +
"/** @return {(number,string)} */ function b() { return 5; }" +
"/** @type {boolean} */ var c = a + b();",
"initializing variable\n" +
"found : (number|string)\n" +
"required: boolean");
}
public void testAdd16() throws Exception {
testTypes("/** @type {(undefined,string)} */ var a = undefined;" +
"/** @type {number} */ var b = 5;" +
"/** @type {boolean} */ var c = a + b;",
"initializing variable\n" +
"found : (number|string)\n" +
"required: boolean");
}
public void testAdd17() throws Exception {
testTypes("/** @type {number} */ var a = 5;" +
"/** @type {(undefined,string)} */ var b = undefined;" +
"/** @type {boolean} */ var c = a + b;",
"initializing variable\n" +
"found : (number|string)\n" +
"required: boolean");
}
public void testAdd18() throws Exception {
testTypes("function f() {};" +
"/** @type {string} */ var a = 'a';" +
"/** @type {number} */ var c = a + f();",
"initializing variable\n" +
"found : string\n" +
"required: number");
}
public void testAdd19() throws Exception {
testTypes("/** @param {number} opt_x\n@param {number} opt_y\n" +
"@return {number} */ function f(opt_x, opt_y) {" +
"return opt_x + opt_y;}");
}
public void testAdd20() throws Exception {
testTypes("/** @param {!Number} opt_x\n@param {!Number} opt_y\n" +
"@return {number} */ function f(opt_x, opt_y) {" +
"return opt_x + opt_y;}");
}
public void testAdd21() throws Exception {
testTypes("/** @param {Number|Boolean} opt_x\n" +
"@param {number|boolean} opt_y\n" +
"@return {number} */ function f(opt_x, opt_y) {" +
"return opt_x + opt_y;}");
}
public void testNumericComparison1() throws Exception {
testTypes("/**@param {number} a*/ function f(a) {return a < 3;}");
}
public void testNumericComparison2() throws Exception {
testTypes("/**@param {!Object} a*/ function f(a) {return a < 3;}",
"left side of numeric comparison\n" +
"found : Object\n" +
"required: number");
}
public void testNumericComparison3() throws Exception {
testTypes("/**@param {string} a*/ function f(a) {return a < 3;}");
}
public void testNumericComparison4() throws Exception {
testTypes("/**@param {(number,undefined)} a*/ " +
"function f(a) {return a < 3;}");
}
public void testNumericComparison5() throws Exception {
testTypes("/**@param {*} a*/ function f(a) {return a < 3;}",
"left side of numeric comparison\n" +
"found : *\n" +
"required: number");
}
public void testNumericComparison6() throws Exception {
testTypes("/**@return {void} */ function foo() { if (3 >= foo()) return; }",
"right side of numeric comparison\n" +
"found : undefined\n" +
"required: number");
}
public void testStringComparison1() throws Exception {
testTypes("/**@param {string} a*/ function f(a) {return a < 'x';}");
}
public void testStringComparison2() throws Exception {
testTypes("/**@param {Object} a*/ function f(a) {return a < 'x';}");
}
public void testStringComparison3() throws Exception {
testTypes("/**@param {number} a*/ function f(a) {return a < 'x';}");
}
public void testStringComparison4() throws Exception {
testTypes("/**@param {string|undefined} a*/ " +
"function f(a) {return a < 'x';}");
}
public void testStringComparison5() throws Exception {
testTypes("/**@param {*} a*/ " +
"function f(a) {return a < 'x';}");
}
public void testStringComparison6() throws Exception {
testTypes("/**@return {void} */ " +
"function foo() { if ('a' >= foo()) return; }",
"right side of comparison\n" +
"found : undefined\n" +
"required: string");
}
public void testValueOfComparison1() throws Exception {
testTypes("/** @constructor */function O() {};" +
"/**@override*/O.prototype.valueOf = function() { return 1; };" +
"/**@param {!O} a\n@param {!O} b*/ function f(a,b) { return a < b; }");
}
public void testValueOfComparison2() throws Exception {
testTypes("/** @constructor */function O() {};" +
"/**@override*/O.prototype.valueOf = function() { return 1; };" +
"/**@param {!O} a\n@param {number} b*/" +
"function f(a,b) { return a < b; }");
}
public void testValueOfComparison3() throws Exception {
testTypes("/** @constructor */function O() {};" +
"/**@override*/O.prototype.toString = function() { return 'o'; };" +
"/**@param {!O} a\n@param {string} b*/" +
"function f(a,b) { return a < b; }");
}
public void testGenericRelationalExpression() throws Exception {
testTypes("/**@param {*} a\n@param {*} b*/ " +
"function f(a,b) {return a < b;}");
}
public void testInstanceof1() throws Exception {
testTypes("function foo(){" +
"if (bar instanceof 3)return;}",
"instanceof requires an object\n" +
"found : number\n" +
"required: Object");
}
public void testInstanceof2() throws Exception {
testTypes("/**@return {void}*/function foo(){" +
"if (foo() instanceof Object)return;}",
"deterministic instanceof yields false\n" +
"found : undefined\n" +
"required: NoObject");
}
public void testInstanceof3() throws Exception {
testTypes("/**@return {*} */function foo(){" +
"if (foo() instanceof Object)return;}");
}
public void testInstanceof4() throws Exception {
testTypes("/**@return {(Object|number)} */function foo(){" +
"if (foo() instanceof Object)return 3;}");
}
public void testInstanceof5() throws Exception {
// No warning for unknown types.
testTypes("/** @return {?} */ function foo(){" +
"if (foo() instanceof Object)return;}");
}
public void testInstanceof6() throws Exception {
testTypes("/**@return {(Array|number)} */function foo(){" +
"if (foo() instanceof Object)return 3;}");
}
public void testInstanceOfReduction3() throws Exception {
testTypes(
"/** \n" +
" * @param {Object} x \n" +
" * @param {Function} y \n" +
" * @return {boolean} \n" +
" */\n" +
"var f = function(x, y) {\n" +
" return x instanceof y;\n" +
"};");
}
public void testScoping1() throws Exception {
testTypes(
"/**@param {string} a*/function foo(a){" +
" /**@param {Array|string} a*/function bar(a){" +
" if (a instanceof Array)return;" +
" }" +
"}");
}
public void testScoping2() throws Exception {
testTypes(
"/** @type number */ var a;" +
"function Foo() {" +
" /** @type string */ var a;" +
"}");
}
public void testScoping3() throws Exception {
testTypes("\n\n/** @type{Number}*/var b;\n/** @type{!String} */var b;",
"variable b redefined with type String, original " +
"definition at [testcode]:3 with type (Number|null)");
}
public void testScoping4() throws Exception {
testTypes("/** @type{Number}*/var b; if (true) /** @type{!String} */var b;",
"variable b redefined with type String, original " +
"definition at [testcode]:1 with type (Number|null)");
}
public void testScoping5() throws Exception {
// multiple definitions are not checked by the type checker but by a
// subsequent pass
testTypes("if (true) var b; var b;");
}
public void testScoping6() throws Exception {
// multiple definitions are not checked by the type checker but by a
// subsequent pass
testTypes("if (true) var b; if (true) var b;");
}
public void testScoping7() throws Exception {
testTypes("/** @constructor */function A() {" +
" /** @type !A */this.a = null;" +
"}",
"assignment to property a of A\n" +
"found : null\n" +
"required: A");
}
public void testScoping8() throws Exception {
testTypes("/** @constructor */function A() {}" +
"/** @constructor */function B() {" +
" /** @type !A */this.a = null;" +
"}",
"assignment to property a of B\n" +
"found : null\n" +
"required: A");
}
public void testScoping9() throws Exception {
testTypes("/** @constructor */function B() {" +
" /** @type !A */this.a = null;" +
"}" +
"/** @constructor */function A() {}",
"assignment to property a of B\n" +
"found : null\n" +
"required: A");
}
public void testScoping10() throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope("var a = function b(){};");
// a declared, b is not
assertTrue(p.scope.isDeclared("a", false));
assertFalse(p.scope.isDeclared("b", false));
// checking that a has the correct assigned type
assertEquals("function (): undefined",
p.scope.getVar("a").getType().toString());
}
public void testScoping11() throws Exception {
// named function expressions create a binding in their body only
// the return is wrong but the assignment is OK since the type of b is ?
testTypes(
"/** @return {number} */var a = function b(){ return b };",
"inconsistent return type\n" +
"found : function (): number\n" +
"required: number");
}
public void testScoping12() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** @type {number} */ F.prototype.bar = 3;" +
"/** @param {!F} f */ function g(f) {" +
" /** @return {string} */" +
" function h() {" +
" return f.bar;" +
" }" +
"}",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testFunctionArguments1() throws Exception {
testFunctionType(
"/** @param {number} a\n@return {string} */" +
"function f(a) {}",
"function (number): string");
}
public void testFunctionArguments2() throws Exception {
testFunctionType(
"/** @param {number} opt_a\n@return {string} */" +
"function f(opt_a) {}",
"function (number=): string");
}
public void testFunctionArguments3() throws Exception {
testFunctionType(
"/** @param {number} b\n@return {string} */" +
"function f(a,b) {}",
"function (?, number): string");
}
public void testFunctionArguments4() throws Exception {
testFunctionType(
"/** @param {number} opt_a\n@return {string} */" +
"function f(a,opt_a) {}",
"function (?, number=): string");
}
public void testFunctionArguments5() throws Exception {
testTypes(
"function a(opt_a,a) {}",
"optional arguments must be at the end");
}
public void testFunctionArguments6() throws Exception {
testTypes(
"function a(var_args,a) {}",
"variable length argument must be last");
}
public void testFunctionArguments7() throws Exception {
testTypes(
"/** @param {number} opt_a\n@return {string} */" +
"function a(a,opt_a,var_args) {}");
}
public void testFunctionArguments8() throws Exception {
testTypes(
"function a(a,opt_a,var_args,b) {}",
"variable length argument must be last");
}
public void testFunctionArguments9() throws Exception {
// testing that only one error is reported
testTypes(
"function a(a,opt_a,var_args,b,c) {}",
"variable length argument must be last");
}
public void testFunctionArguments10() throws Exception {
// testing that only one error is reported
testTypes(
"function a(a,opt_a,b,c) {}",
"optional arguments must be at the end");
}
public void testFunctionArguments11() throws Exception {
testTypes(
"function a(a,opt_a,b,c,var_args,d) {}",
"optional arguments must be at the end");
}
public void testFunctionArguments12() throws Exception {
testTypes("/** @param foo {String} */function bar(baz){}",
"parameter foo does not appear in bar's parameter list");
}
public void testFunctionArguments13() throws Exception {
// verifying that the argument type have non-inferable types
testTypes(
"/** @return {boolean} */ function u() { return true; }" +
"/** @param {boolean} b\n@return {?boolean} */" +
"function f(b) { if (u()) { b = null; } return b; }",
"assignment\n" +
"found : null\n" +
"required: boolean");
}
public void testFunctionArguments14() throws Exception {
testTypes(
"/**\n" +
" * @param {string} x\n" +
" * @param {number} opt_y\n" +
" * @param {boolean} var_args\n" +
" */ function f(x, opt_y, var_args) {}" +
"f('3'); f('3', 2); f('3', 2, true); f('3', 2, true, false);");
}
public void testFunctionArguments15() throws Exception {
testTypes(
"/** @param {?function(*)} f */" +
"function g(f) { f(1, 2); }",
"Function f: called with 2 argument(s). " +
"Function requires at least 1 argument(s) " +
"and no more than 1 argument(s).");
}
public void testFunctionArguments16() throws Exception {
testTypes(
"/** @param {...number} var_args */" +
"function g(var_args) {} g(1, true);",
"actual parameter 2 of g does not match formal parameter\n" +
"found : boolean\n" +
"required: (number|undefined)");
}
public void testFunctionArguments17() throws Exception {
testClosureTypesMultipleWarnings(
"/** @param {booool|string} x */" +
"function f(x) { g(x) }" +
"/** @param {number} x */" +
"function g(x) {}",
Lists.newArrayList(
"Bad type annotation. Unknown type booool",
"actual parameter 1 of g does not match formal parameter\n" +
"found : (booool|null|string)\n" +
"required: number"));
}
public void testPrintFunctionName1() throws Exception {
// Ensures that the function name is pretty.
testTypes(
"var goog = {}; goog.run = function(f) {};" +
"goog.run();",
"Function goog.run: called with 0 argument(s). " +
"Function requires at least 1 argument(s) " +
"and no more than 1 argument(s).");
}
public void testPrintFunctionName2() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {}; " +
"Foo.prototype.run = function(f) {};" +
"(new Foo).run();",
"Function Foo.prototype.run: called with 0 argument(s). " +
"Function requires at least 1 argument(s) " +
"and no more than 1 argument(s).");
}
public void testFunctionInference1() throws Exception {
testFunctionType(
"function f(a) {}",
"function (?): undefined");
}
public void testFunctionInference2() throws Exception {
testFunctionType(
"function f(a,b) {}",
"function (?, ?): undefined");
}
public void testFunctionInference3() throws Exception {
testFunctionType(
"function f(var_args) {}",
"function (...[?]): undefined");
}
public void testFunctionInference4() throws Exception {
testFunctionType(
"function f(a,b,c,var_args) {}",
"function (?, ?, ?, ...[?]): undefined");
}
public void testFunctionInference5() throws Exception {
testFunctionType(
"/** @this Date\n@return {string} */function f(a) {}",
"function (this:Date, ?): string");
}
public void testFunctionInference6() throws Exception {
testFunctionType(
"/** @this Date\n@return {string} */function f(opt_a) {}",
"function (this:Date, ?=): string");
}
public void testFunctionInference7() throws Exception {
testFunctionType(
"/** @this Date */function f(a,b,c,var_args) {}",
"function (this:Date, ?, ?, ?, ...[?]): undefined");
}
public void testFunctionInference8() throws Exception {
testFunctionType(
"function f() {}",
"function (): undefined");
}
public void testFunctionInference9() throws Exception {
testFunctionType(
"var f = function() {};",
"function (): undefined");
}
public void testFunctionInference10() throws Exception {
testFunctionType(
"/** @this Date\n@param {boolean} b\n@return {string} */" +
"var f = function(a,b) {};",
"function (this:Date, ?, boolean): string");
}
public void testFunctionInference11() throws Exception {
testFunctionType(
"var goog = {};" +
"/** @return {number}*/goog.f = function(){};",
"goog.f",
"function (): number");
}
public void testFunctionInference12() throws Exception {
testFunctionType(
"var goog = {};" +
"goog.f = function(){};",
"goog.f",
"function (): undefined");
}
public void testFunctionInference13() throws Exception {
testFunctionType(
"var goog = {};" +
"/** @constructor */ goog.Foo = function(){};" +
"/** @param {!goog.Foo} f */function eatFoo(f){};",
"eatFoo",
"function (goog.Foo): undefined");
}
public void testFunctionInference14() throws Exception {
testFunctionType(
"var goog = {};" +
"/** @constructor */ goog.Foo = function(){};" +
"/** @return {!goog.Foo} */function eatFoo(){ return new goog.Foo; };",
"eatFoo",
"function (): goog.Foo");
}
public void testFunctionInference15() throws Exception {
testFunctionType(
"/** @constructor */ function f() {};" +
"f.prototype.foo = function(){};",
"f.prototype.foo",
"function (this:f): undefined");
}
public void testFunctionInference16() throws Exception {
testFunctionType(
"/** @constructor */ function f() {};" +
"f.prototype.foo = function(){};",
"(new f).foo",
"function (this:f): undefined");
}
public void testFunctionInference17() throws Exception {
testFunctionType(
"/** @constructor */ function f() {}" +
"function abstractMethod() {}" +
"/** @param {number} x */ f.prototype.foo = abstractMethod;",
"(new f).foo",
"function (this:f, number): ?");
}
public void testFunctionInference18() throws Exception {
testFunctionType(
"var goog = {};" +
"/** @this {Date} */ goog.eatWithDate;",
"goog.eatWithDate",
"function (this:Date): ?");
}
public void testFunctionInference19() throws Exception {
testFunctionType(
"/** @param {string} x */ var f;",
"f",
"function (string): ?");
}
public void testFunctionInference20() throws Exception {
testFunctionType(
"/** @this {Date} */ var f;",
"f",
"function (this:Date): ?");
}
public void testFunctionInference21() throws Exception {
testTypes(
"var f = function() { throw 'x' };" +
"/** @return {boolean} */ var g = f;");
testFunctionType(
"var f = function() { throw 'x' };",
"f",
"function (): ?");
}
public void testFunctionInference22() throws Exception {
testTypes(
"/** @type {!Function} */ var f = function() { g(this); };" +
"/** @param {boolean} x */ var g = function(x) {};");
}
public void testFunctionInference23() throws Exception {
// We want to make sure that 'prop' isn't declared on all objects.
testTypes(
"/** @type {!Function} */ var f = function() {\n" +
" /** @type {number} */ this.prop = 3;\n" +
"};" +
"/**\n" +
" * @param {Object} x\n" +
" * @return {string}\n" +
" */ var g = function(x) { return x.prop; };");
}
public void testInnerFunction1() throws Exception {
testTypes(
"function f() {" +
" /** @type {number} */ var x = 3;\n" +
" function g() { x = null; }" +
" return x;" +
"}",
"assignment\n" +
"found : null\n" +
"required: number");
}
public void testInnerFunction2() throws Exception {
testTypes(
"/** @return {number} */\n" +
"function f() {" +
" var x = null;\n" +
" function g() { x = 3; }" +
" g();" +
" return x;" +
"}",
"inconsistent return type\n" +
"found : (null|number)\n" +
"required: number");
}
public void testInnerFunction3() throws Exception {
testTypes(
"var x = null;" +
"/** @return {number} */\n" +
"function f() {" +
" x = 3;\n" +
" /** @return {number} */\n" +
" function g() { x = true; return x; }" +
" return x;" +
"}",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testInnerFunction4() throws Exception {
testTypes(
"var x = null;" +
"/** @return {number} */\n" +
"function f() {" +
" x = '3';\n" +
" /** @return {number} */\n" +
" function g() { x = 3; return x; }" +
" return x;" +
"}",
"inconsistent return type\n" +
"found : string\n" +
"required: number");
}
public void testInnerFunction5() throws Exception {
testTypes(
"/** @return {number} */\n" +
"function f() {" +
" var x = 3;\n" +
" /** @return {number} */" +
" function g() { var x = 3;x = true; return x; }" +
" return x;" +
"}",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testInnerFunction6() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"function f() {" +
" var x = 0 || function() {};\n" +
" function g() { if (goog.isFunction(x)) { x(1); } }" +
" g();" +
"}",
"Function x: called with 1 argument(s). " +
"Function requires at least 0 argument(s) " +
"and no more than 0 argument(s).");
}
public void testInnerFunction7() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"function f() {" +
" /** @type {number|function()} */" +
" var x = 0 || function() {};\n" +
" function g() { if (goog.isFunction(x)) { x(1); } }" +
" g();" +
"}",
"Function x: called with 1 argument(s). " +
"Function requires at least 0 argument(s) " +
"and no more than 0 argument(s).");
}
public void testInnerFunction8() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"function f() {" +
" function x() {};\n" +
" function g() { if (goog.isFunction(x)) { x(1); } }" +
" g();" +
"}",
"Function x: called with 1 argument(s). " +
"Function requires at least 0 argument(s) " +
"and no more than 0 argument(s).");
}
public void testInnerFunction9() throws Exception {
testTypes(
"function f() {" +
" var x = 3;\n" +
" function g() { x = null; };\n" +
" function h() { return x == null; }" +
" return h();" +
"}");
}
public void testInnerFunction10() throws Exception {
testTypes(
"function f() {" +
" /** @type {?number} */ var x = null;" +
" /** @return {string} */" +
" function g() {" +
" if (!x) {" +
" x = 1;" +
" }" +
" return x;" +
" }" +
"}",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testInnerFunction11() throws Exception {
// TODO(nicksantos): This is actually bad inference, because
// h sets x to null. We should fix this, but for now we do it
// this way so that we don't break existing binaries. We will
// need to change TypeInference#isUnflowable to fix this.
testTypes(
"function f() {" +
" /** @type {?number} */ var x = null;" +
" /** @return {number} */" +
" function g() {" +
" x = 1;" +
" h();" +
" return x;" +
" }" +
" function h() {" +
" x = null;" +
" }" +
"}");
}
public void testAbstractMethodHandling1() throws Exception {
testTypes(
"/** @type {Function} */ var abstractFn = function() {};" +
"abstractFn(1);");
}
public void testAbstractMethodHandling2() throws Exception {
testTypes(
"var abstractFn = function() {};" +
"abstractFn(1);",
"Function abstractFn: called with 1 argument(s). " +
"Function requires at least 0 argument(s) " +
"and no more than 0 argument(s).");
}
public void testAbstractMethodHandling3() throws Exception {
testTypes(
"var goog = {};" +
"/** @type {Function} */ goog.abstractFn = function() {};" +
"goog.abstractFn(1);");
}
public void testAbstractMethodHandling4() throws Exception {
testTypes(
"var goog = {};" +
"goog.abstractFn = function() {};" +
"goog.abstractFn(1);",
"Function goog.abstractFn: called with 1 argument(s). " +
"Function requires at least 0 argument(s) " +
"and no more than 0 argument(s).");
}
public void testAbstractMethodHandling5() throws Exception {
testTypes(
"/** @type {!Function} */ var abstractFn = function() {};" +
"/** @param {number} x */ var f = abstractFn;" +
"f('x');",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testAbstractMethodHandling6() throws Exception {
testTypes(
"var goog = {};" +
"/** @type {Function} */ goog.abstractFn = function() {};" +
"/** @param {number} x */ goog.f = abstractFn;" +
"goog.f('x');",
"actual parameter 1 of goog.f does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testMethodInference1() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** @return {number} */ F.prototype.foo = function() { return 3; };" +
"/** @constructor \n * @extends {F} */ " +
"function G() {}" +
"/** @override */ G.prototype.foo = function() { return true; };",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testMethodInference2() throws Exception {
testTypes(
"var goog = {};" +
"/** @constructor */ goog.F = function() {};" +
"/** @return {number} */ goog.F.prototype.foo = " +
" function() { return 3; };" +
"/** @constructor \n * @extends {goog.F} */ " +
"goog.G = function() {};" +
"/** @override */ goog.G.prototype.foo = function() { return true; };",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testMethodInference3() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** @param {boolean} x \n * @return {number} */ " +
"F.prototype.foo = function(x) { return 3; };" +
"/** @constructor \n * @extends {F} */ " +
"function G() {}" +
"/** @override */ " +
"G.prototype.foo = function(x) { return x; };",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testMethodInference4() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** @param {boolean} x \n * @return {number} */ " +
"F.prototype.foo = function(x) { return 3; };" +
"/** @constructor \n * @extends {F} */ " +
"function G() {}" +
"/** @override */ " +
"G.prototype.foo = function(y) { return y; };",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testMethodInference5() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** @param {number} x \n * @return {string} */ " +
"F.prototype.foo = function(x) { return 'x'; };" +
"/** @constructor \n * @extends {F} */ " +
"function G() {}" +
"/** @type {number} */ G.prototype.num = 3;" +
"/** @override */ " +
"G.prototype.foo = function(y) { return this.num + y; };",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testMethodInference6() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** @param {number} x */ F.prototype.foo = function(x) { };" +
"/** @constructor \n * @extends {F} */ " +
"function G() {}" +
"/** @override */ G.prototype.foo = function() { };" +
"(new G()).foo(1);");
}
public void testMethodInference7() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"F.prototype.foo = function() { };" +
"/** @constructor \n * @extends {F} */ " +
"function G() {}" +
"/** @override */ G.prototype.foo = function(x, y) { };",
"mismatch of the foo property type and the type of the property " +
"it overrides from superclass F\n" +
"original: function (this:F): undefined\n" +
"override: function (this:G, ?, ?): undefined");
}
public void testMethodInference8() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"F.prototype.foo = function() { };" +
"/** @constructor \n * @extends {F} */ " +
"function G() {}" +
"/** @override */ " +
"G.prototype.foo = function(opt_b, var_args) { };" +
"(new G()).foo(1, 2, 3);");
}
public void testMethodInference9() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"F.prototype.foo = function() { };" +
"/** @constructor \n * @extends {F} */ " +
"function G() {}" +
"/** @override */ " +
"G.prototype.foo = function(var_args, opt_b) { };",
"variable length argument must be last");
}
public void testStaticMethodDeclaration1() throws Exception {
testTypes(
"/** @constructor */ function F() { F.foo(true); }" +
"/** @param {number} x */ F.foo = function(x) {};",
"actual parameter 1 of F.foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testStaticMethodDeclaration2() throws Exception {
testTypes(
"var goog = goog || {}; function f() { goog.foo(true); }" +
"/** @param {number} x */ goog.foo = function(x) {};",
"actual parameter 1 of goog.foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testStaticMethodDeclaration3() throws Exception {
testTypes(
"var goog = goog || {}; function f() { goog.foo(true); }" +
"goog.foo = function() {};",
"Function goog.foo: called with 1 argument(s). Function requires " +
"at least 0 argument(s) and no more than 0 argument(s).");
}
public void testDuplicateStaticMethodDecl1() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @param {number} x */ goog.foo = function(x) {};" +
"/** @param {number} x */ goog.foo = function(x) {};",
"variable goog.foo redefined with type function (number): undefined, " +
"original definition at [testcode]:1 " +
"with type function (number): undefined");
}
public void testDuplicateStaticMethodDecl2() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @param {number} x */ goog.foo = function(x) {};" +
"/** @param {number} x \n * @suppress {duplicate} */ " +
"goog.foo = function(x) {};");
}
public void testDuplicateStaticMethodDecl3() throws Exception {
testTypes(
"var goog = goog || {};" +
"goog.foo = function(x) {};" +
"goog.foo = function(x) {};");
}
public void testDuplicateStaticMethodDecl4() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @type {Function} */ goog.foo = function(x) {};" +
"goog.foo = function(x) {};");
}
public void testDuplicateStaticMethodDecl5() throws Exception {
testTypes(
"var goog = goog || {};" +
"goog.foo = function(x) {};" +
"/** @return {undefined} */ goog.foo = function(x) {};",
"variable goog.foo redefined with type function (?): undefined, " +
"original definition at [testcode]:1 with type " +
"function (?): undefined");
}
public void testDuplicateStaticPropertyDecl1() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @type {Foo} */ goog.foo;" +
"/** @type {Foo} */ goog.foo;" +
"/** @constructor */ function Foo() {}");
}
public void testDuplicateStaticPropertyDecl2() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @type {Foo} */ goog.foo;" +
"/** @type {Foo} \n * @suppress {duplicate} */ goog.foo;" +
"/** @constructor */ function Foo() {}");
}
public void testDuplicateStaticPropertyDecl3() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @type {!Foo} */ goog.foo;" +
"/** @type {string} */ goog.foo;" +
"/** @constructor */ function Foo() {}",
"variable goog.foo redefined with type string, " +
"original definition at [testcode]:1 with type Foo");
}
public void testDuplicateStaticPropertyDecl4() throws Exception {
testClosureTypesMultipleWarnings(
"var goog = goog || {};" +
"/** @type {!Foo} */ goog.foo;" +
"/** @type {string} */ goog.foo = 'x';" +
"/** @constructor */ function Foo() {}",
Lists.newArrayList(
"assignment to property foo of goog\n" +
"found : string\n" +
"required: Foo",
"variable goog.foo redefined with type string, " +
"original definition at [testcode]:1 with type Foo"));
}
public void testDuplicateStaticPropertyDecl5() throws Exception {
testClosureTypesMultipleWarnings(
"var goog = goog || {};" +
"/** @type {!Foo} */ goog.foo;" +
"/** @type {string}\n * @suppress {duplicate} */ goog.foo = 'x';" +
"/** @constructor */ function Foo() {}",
Lists.newArrayList(
"assignment to property foo of goog\n" +
"found : string\n" +
"required: Foo",
"variable goog.foo redefined with type string, " +
"original definition at [testcode]:1 with type Foo"));
}
public void testDuplicateStaticPropertyDecl6() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @type {string} */ goog.foo = 'y';" +
"/** @type {string}\n * @suppress {duplicate} */ goog.foo = 'x';");
}
public void testDuplicateStaticPropertyDecl7() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @param {string} x */ goog.foo;" +
"/** @type {function(string)} */ goog.foo;");
}
public void testDuplicateStaticPropertyDecl8() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @return {EventCopy} */ goog.foo;" +
"/** @constructor */ function EventCopy() {}" +
"/** @return {EventCopy} */ goog.foo;");
}
public void testDuplicateStaticPropertyDecl9() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @return {EventCopy} */ goog.foo;" +
"/** @return {EventCopy} */ goog.foo;" +
"/** @constructor */ function EventCopy() {}");
}
public void testDuplicateStaticPropertyDec20() throws Exception {
testTypes(
"/**\n" +
" * @fileoverview\n" +
" * @suppress {duplicate}\n" +
" */" +
"var goog = goog || {};" +
"/** @type {string} */ goog.foo = 'y';" +
"/** @type {string} */ goog.foo = 'x';");
}
public void testDuplicateLocalVarDecl() throws Exception {
testClosureTypesMultipleWarnings(
"/** @param {number} x */\n" +
"function f(x) { /** @type {string} */ var x = ''; }",
Lists.newArrayList(
"variable x redefined with type string, original definition" +
" at [testcode]:2 with type number",
"initializing variable\n" +
"found : string\n" +
"required: number"));
}
public void testDuplicateInstanceMethod1() throws Exception {
// If there's no jsdoc on the methods, then we treat them like
// any other inferred properties.
testTypes(
"/** @constructor */ function F() {}" +
"F.prototype.bar = function() {};" +
"F.prototype.bar = function() {};");
}
public void testDuplicateInstanceMethod2() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** jsdoc */ F.prototype.bar = function() {};" +
"/** jsdoc */ F.prototype.bar = function() {};",
"variable F.prototype.bar redefined with type " +
"function (this:F): undefined, original definition at " +
"[testcode]:1 with type function (this:F): undefined");
}
public void testDuplicateInstanceMethod3() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"F.prototype.bar = function() {};" +
"/** jsdoc */ F.prototype.bar = function() {};",
"variable F.prototype.bar redefined with type " +
"function (this:F): undefined, original definition at " +
"[testcode]:1 with type function (this:F): undefined");
}
public void testDuplicateInstanceMethod4() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** jsdoc */ F.prototype.bar = function() {};" +
"F.prototype.bar = function() {};");
}
public void testDuplicateInstanceMethod5() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** jsdoc \n * @return {number} */ F.prototype.bar = function() {" +
" return 3;" +
"};" +
"/** jsdoc \n * @suppress {duplicate} */ " +
"F.prototype.bar = function() { return ''; };",
"inconsistent return type\n" +
"found : string\n" +
"required: number");
}
public void testDuplicateInstanceMethod6() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** jsdoc \n * @return {number} */ F.prototype.bar = function() {" +
" return 3;" +
"};" +
"/** jsdoc \n * @return {string} * \n @suppress {duplicate} */ " +
"F.prototype.bar = function() { return ''; };",
"assignment to property bar of F.prototype\n" +
"found : function (this:F): string\n" +
"required: function (this:F): number");
}
public void testStubFunctionDeclaration1() throws Exception {
testFunctionType(
"/** @constructor */ function f() {};" +
"/** @param {number} x \n * @param {string} y \n" +
" * @return {number} */ f.prototype.foo;",
"(new f).foo",
"function (this:f, number, string): number");
}
public void testStubFunctionDeclaration2() throws Exception {
testExternFunctionType(
// externs
"/** @constructor */ function f() {};" +
"/** @constructor \n * @extends {f} */ f.subclass;",
"f.subclass",
"function (new:f.subclass): ?");
}
public void testStubFunctionDeclaration3() throws Exception {
testFunctionType(
"/** @constructor */ function f() {};" +
"/** @return {undefined} */ f.foo;",
"f.foo",
"function (): undefined");
}
public void testStubFunctionDeclaration4() throws Exception {
testFunctionType(
"/** @constructor */ function f() { " +
" /** @return {number} */ this.foo;" +
"}",
"(new f).foo",
"function (this:f): number");
}
public void testStubFunctionDeclaration5() throws Exception {
testFunctionType(
"/** @constructor */ function f() { " +
" /** @type {Function} */ this.foo;" +
"}",
"(new f).foo",
createNullableType(U2U_CONSTRUCTOR_TYPE).toString());
}
public void testStubFunctionDeclaration6() throws Exception {
testFunctionType(
"/** @constructor */ function f() {} " +
"/** @type {Function} */ f.prototype.foo;",
"(new f).foo",
createNullableType(U2U_CONSTRUCTOR_TYPE).toString());
}
public void testStubFunctionDeclaration7() throws Exception {
testFunctionType(
"/** @constructor */ function f() {} " +
"/** @type {Function} */ f.prototype.foo = function() {};",
"(new f).foo",
createNullableType(U2U_CONSTRUCTOR_TYPE).toString());
}
public void testStubFunctionDeclaration8() throws Exception {
testFunctionType(
"/** @type {Function} */ var f = function() {}; ",
"f",
createNullableType(U2U_CONSTRUCTOR_TYPE).toString());
}
public void testStubFunctionDeclaration9() throws Exception {
testFunctionType(
"/** @type {function():number} */ var f; ",
"f",
"function (): number");
}
public void testStubFunctionDeclaration10() throws Exception {
testFunctionType(
"/** @type {function(number):number} */ var f = function(x) {};",
"f",
"function (number): number");
}
public void testNestedFunctionInference1() throws Exception {
String nestedAssignOfFooAndBar =
"/** @constructor */ function f() {};" +
"f.prototype.foo = f.prototype.bar = function(){};";
testFunctionType(nestedAssignOfFooAndBar, "(new f).bar",
"function (this:f): undefined");
}
/**
* Tests the type of a function definition. The function defined by
* {@code functionDef} should be named {@code "f"}.
*/
private void testFunctionType(String functionDef, String functionType)
throws Exception {
testFunctionType(functionDef, "f", functionType);
}
/**
* Tests the type of a function definition. The function defined by
* {@code functionDef} should be named {@code functionName}.
*/
private void testFunctionType(String functionDef, String functionName,
String functionType) throws Exception {
// using the variable initialization check to verify the function's type
testTypes(
functionDef +
"/** @type number */var a=" + functionName + ";",
"initializing variable\n" +
"found : " + functionType + "\n" +
"required: number");
}
/**
* Tests the type of a function definition in externs.
* The function defined by {@code functionDef} should be
* named {@code functionName}.
*/
private void testExternFunctionType(String functionDef, String functionName,
String functionType) throws Exception {
testTypes(
functionDef,
"/** @type number */var a=" + functionName + ";",
"initializing variable\n" +
"found : " + functionType + "\n" +
"required: number", false);
}
public void testTypeRedefinition() throws Exception {
testClosureTypesMultipleWarnings("a={};/**@enum {string}*/ a.A = {ZOR:'b'};"
+ "/** @constructor */ a.A = function() {}",
Lists.newArrayList(
"variable a.A redefined with type function (new:a.A): undefined, " +
"original definition at [testcode]:1 with type enum{a.A}",
"assignment to property A of a\n" +
"found : function (new:a.A): undefined\n" +
"required: enum{a.A}"));
}
public void testIn1() throws Exception {
testTypes("'foo' in Object");
}
public void testIn2() throws Exception {
testTypes("3 in Object");
}
public void testIn3() throws Exception {
testTypes("undefined in Object");
}
public void testIn4() throws Exception {
testTypes("Date in Object",
"left side of 'in'\n" +
"found : function (new:Date, ?=, ?=, ?=, ?=, ?=, ?=, ?=): string\n" +
"required: string");
}
public void testIn5() throws Exception {
testTypes("'x' in null",
"'in' requires an object\n" +
"found : null\n" +
"required: Object");
}
public void testIn6() throws Exception {
testTypes(
"/** @param {number} x */" +
"function g(x) {}" +
"g(1 in {});",
"actual parameter 1 of g does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testIn7() throws Exception {
// Make sure we do inference in the 'in' expression.
testTypes(
"/**\n" +
" * @param {number} x\n" +
" * @return {number}\n" +
" */\n" +
"function g(x) { return 5; }" +
"function f() {" +
" var x = {};" +
" x.foo = '3';" +
" return g(x.foo) in {};" +
"}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testForIn1() throws Exception {
testTypes(
"/** @param {boolean} x */ function f(x) {}" +
"for (var k in {}) {" +
" f(k);" +
"}",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: boolean");
}
public void testForIn2() throws Exception {
testTypes(
"/** @param {boolean} x */ function f(x) {}" +
"/** @enum {string} */ var E = {FOO: 'bar'};" +
"/** @type {Object.<E, string>} */ var obj = {};" +
"var k = null;" +
"for (k in obj) {" +
" f(k);" +
"}",
"actual parameter 1 of f does not match formal parameter\n" +
"found : E.<string>\n" +
"required: boolean");
}
public void testForIn3() throws Exception {
testTypes(
"/** @param {boolean} x */ function f(x) {}" +
"/** @type {Object.<number>} */ var obj = {};" +
"for (var k in obj) {" +
" f(obj[k]);" +
"}",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: boolean");
}
public void testForIn4() throws Exception {
testTypes(
"/** @param {boolean} x */ function f(x) {}" +
"/** @enum {string} */ var E = {FOO: 'bar'};" +
"/** @type {Object.<E, Array>} */ var obj = {};" +
"for (var k in obj) {" +
" f(obj[k]);" +
"}",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (Array|null)\n" +
"required: boolean");
}
public void testForIn5() throws Exception {
testTypes(
"/** @param {boolean} x */ function f(x) {}" +
"/** @constructor */ var E = function(){};" +
"/** @type {Object.<E, number>} */ var obj = {};" +
"for (var k in obj) {" +
" f(k);" +
"}",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: boolean");
}
// TODO(nicksantos): change this to something that makes sense.
// public void testComparison1() throws Exception {
// testTypes("/**@type null */var a;" +
// "/**@type !Date */var b;" +
// "if (a==b) {}",
// "condition always evaluates to false\n" +
// "left : null\n" +
// "right: Date");
// }
public void testComparison2() throws Exception {
testTypes("/**@type number*/var a;" +
"/**@type !Date */var b;" +
"if (a!==b) {}",
"condition always evaluates to true\n" +
"left : number\n" +
"right: Date");
}
public void testComparison3() throws Exception {
// Since null == undefined in JavaScript, this code is reasonable.
testTypes("/** @type {(Object,undefined)} */var a;" +
"var b = a == null");
}
public void testComparison4() throws Exception {
testTypes("/** @type {(!Object,undefined)} */var a;" +
"/** @type {!Object} */var b;" +
"var c = a == b");
}
public void testComparison5() throws Exception {
testTypes("/** @type null */var a;" +
"/** @type null */var b;" +
"a == b",
"condition always evaluates to true\n" +
"left : null\n" +
"right: null");
}
public void testComparison6() throws Exception {
testTypes("/** @type null */var a;" +
"/** @type null */var b;" +
"a != b",
"condition always evaluates to false\n" +
"left : null\n" +
"right: null");
}
public void testComparison7() throws Exception {
testTypes("var a;" +
"var b;" +
"a == b",
"condition always evaluates to true\n" +
"left : undefined\n" +
"right: undefined");
}
public void testComparison8() throws Exception {
testTypes("/** @type {Array.<string>} */ var a = [];" +
"a[0] == null || a[1] == undefined");
}
public void testComparison9() throws Exception {
testTypes("/** @type {Array.<undefined>} */ var a = [];" +
"a[0] == null",
"condition always evaluates to true\n" +
"left : undefined\n" +
"right: null");
}
public void testComparison10() throws Exception {
testTypes("/** @type {Array.<undefined>} */ var a = [];" +
"a[0] === null");
}
public void testComparison11() throws Exception {
testTypes(
"(function(){}) == 'x'",
"condition always evaluates to false\n" +
"left : function (): undefined\n" +
"right: string");
}
public void testComparison12() throws Exception {
testTypes(
"(function(){}) == 3",
"condition always evaluates to false\n" +
"left : function (): undefined\n" +
"right: number");
}
public void testComparison13() throws Exception {
testTypes(
"(function(){}) == false",
"condition always evaluates to false\n" +
"left : function (): undefined\n" +
"right: boolean");
}
public void testComparison14() throws Exception {
testTypes("/** @type {function((Array|string), Object): number} */" +
"function f(x, y) { return x === y; }",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testComparison15() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @constructor */ function F() {}" +
"/**\n" +
" * @param {number} x\n" +
" * @constructor\n" +
" * @extends {F}\n" +
" */\n" +
"function G(x) {}\n" +
"goog.inherits(G, F);\n" +
"/**\n" +
" * @param {number} x\n" +
" * @constructor\n" +
" * @extends {G}\n" +
" */\n" +
"function H(x) {}\n" +
"goog.inherits(H, G);\n" +
"/** @param {G} x */" +
"function f(x) { return x.constructor === H; }",
null);
}
public void testDeleteOperator1() throws Exception {
testTypes(
"var x = {};" +
"/** @return {string} */ function f() { return delete x['a']; }",
"inconsistent return type\n" +
"found : boolean\n" +
"required: string");
}
public void testDeleteOperator2() throws Exception {
testTypes(
"var obj = {};" +
"/** \n" +
" * @param {string} x\n" +
" * @return {Object} */ function f(x) { return obj; }" +
"/** @param {?number} x */ function g(x) {" +
" if (x) { delete f(x)['a']; }" +
"}",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testEnumStaticMethod1() throws Exception {
testTypes(
"/** @enum */ var Foo = {AAA: 1};" +
"/** @param {number} x */ Foo.method = function(x) {};" +
"Foo.method(true);",
"actual parameter 1 of Foo.method does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testEnumStaticMethod2() throws Exception {
testTypes(
"/** @enum */ var Foo = {AAA: 1};" +
"/** @param {number} x */ Foo.method = function(x) {};" +
"function f() { Foo.method(true); }",
"actual parameter 1 of Foo.method does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testEnum1() throws Exception {
testTypes("/**@enum*/var a={BB:1,CC:2};\n" +
"/**@type {a}*/var d;d=a.BB;");
}
public void testEnum2() throws Exception {
testTypes("/**@enum*/var a={b:1}",
"enum key b must be a syntactic constant");
}
public void testEnum3() throws Exception {
testTypes("/**@enum*/var a={BB:1,BB:2}",
"variable a.BB redefined with type a.<number>, " +
"original definition at [testcode]:1 with type a.<number>");
}
public void testEnum4() throws Exception {
testTypes("/**@enum*/var a={BB:'string'}",
"assignment to property BB of enum{a}\n" +
"found : string\n" +
"required: number");
}
public void testEnum5() throws Exception {
testTypes("/**@enum {String}*/var a={BB:'string'}",
"assignment to property BB of enum{a}\n" +
"found : string\n" +
"required: (String|null)");
}
public void testEnum6() throws Exception {
testTypes("/**@enum*/var a={BB:1,CC:2};\n/**@type {!Array}*/var d;d=a.BB;",
"assignment\n" +
"found : a.<number>\n" +
"required: Array");
}
public void testEnum7() throws Exception {
testTypes("/** @enum */var a={AA:1,BB:2,CC:3};" +
"/** @type a */var b=a.D;",
"element D does not exist on this enum");
}
public void testEnum8() throws Exception {
testClosureTypesMultipleWarnings("/** @enum */var a=8;",
Lists.newArrayList(
"enum initializer must be an object literal or an enum",
"initializing variable\n" +
"found : number\n" +
"required: enum{a}"));
}
public void testEnum9() throws Exception {
testClosureTypesMultipleWarnings(
"var goog = {};" +
"/** @enum */goog.a=8;",
Lists.newArrayList(
"assignment to property a of goog\n" +
"found : number\n" +
"required: enum{goog.a}",
"enum initializer must be an object literal or an enum"));
}
public void testEnum10() throws Exception {
testTypes(
"/** @enum {number} */" +
"goog.K = { A : 3 };");
}
public void testEnum11() throws Exception {
testTypes(
"/** @enum {number} */" +
"goog.K = { 502 : 3 };");
}
public void testEnum12() throws Exception {
testTypes(
"/** @enum {number} */ var a = {};" +
"/** @enum */ var b = a;");
}
public void testEnum13() throws Exception {
testTypes(
"/** @enum {number} */ var a = {};" +
"/** @enum {string} */ var b = a;",
"incompatible enum element types\n" +
"found : number\n" +
"required: string");
}
public void testEnum14() throws Exception {
testTypes(
"/** @enum {number} */ var a = {FOO:5};" +
"/** @enum */ var b = a;" +
"var c = b.FOO;");
}
public void testEnum15() throws Exception {
testTypes(
"/** @enum {number} */ var a = {FOO:5};" +
"/** @enum */ var b = a;" +
"var c = b.BAR;",
"element BAR does not exist on this enum");
}
public void testEnum16() throws Exception {
testTypes("var goog = {};" +
"/**@enum*/goog .a={BB:1,BB:2}",
"variable goog.a.BB redefined with type goog.a.<number>, " +
"original definition at [testcode]:1 with type goog.a.<number>");
}
public void testEnum17() throws Exception {
testTypes("var goog = {};" +
"/**@enum*/goog.a={BB:'string'}",
"assignment to property BB of enum{goog.a}\n" +
"found : string\n" +
"required: number");
}
public void testEnum18() throws Exception {
testTypes("/**@enum*/ var E = {A: 1, B: 2};" +
"/** @param {!E} x\n@return {number} */\n" +
"var f = function(x) { return x; };");
}
public void testEnum19() throws Exception {
testTypes("/**@enum*/ var E = {A: 1, B: 2};" +
"/** @param {number} x\n@return {!E} */\n" +
"var f = function(x) { return x; };",
"inconsistent return type\n" +
"found : number\n" +
"required: E.<number>");
}
public void testEnum20() throws Exception {
testTypes("/**@enum*/ var E = {A: 1, B: 2}; var x = []; x[E.A] = 0;");
}
public void testEnum21() throws Exception {
Node n = parseAndTypeCheck(
"/** @enum {string} */ var E = {A : 'a', B : 'b'};\n" +
"/** @param {!E} x\n@return {!E} */ function f(x) { return x; }");
Node nodeX = n.getLastChild().getLastChild().getLastChild().getLastChild();
JSType typeE = nodeX.getJSType();
assertFalse(typeE.isObject());
assertFalse(typeE.isNullable());
}
public void testEnum22() throws Exception {
testTypes("/**@enum*/ var E = {A: 1, B: 2};" +
"/** @param {E} x \n* @return {number} */ function f(x) {return x}");
}
public void testEnum23() throws Exception {
testTypes("/**@enum*/ var E = {A: 1, B: 2};" +
"/** @param {E} x \n* @return {string} */ function f(x) {return x}",
"inconsistent return type\n" +
"found : E.<number>\n" +
"required: string");
}
public void testEnum24() throws Exception {
testTypes("/**@enum {Object} */ var E = {A: {}};" +
"/** @param {E} x \n* @return {!Object} */ function f(x) {return x}",
"inconsistent return type\n" +
"found : E.<(Object|null)>\n" +
"required: Object");
}
public void testEnum25() throws Exception {
testTypes("/**@enum {!Object} */ var E = {A: {}};" +
"/** @param {E} x \n* @return {!Object} */ function f(x) {return x}");
}
public void testEnum26() throws Exception {
testTypes("var a = {}; /**@enum*/ a.B = {A: 1, B: 2};" +
"/** @param {a.B} x \n* @return {number} */ function f(x) {return x}");
}
public void testEnum27() throws Exception {
// x is unknown
testTypes("/** @enum */ var A = {B: 1, C: 2}; " +
"function f(x) { return A == x; }");
}
public void testEnum28() throws Exception {
// x is unknown
testTypes("/** @enum */ var A = {B: 1, C: 2}; " +
"function f(x) { return A.B == x; }");
}
public void testEnum29() throws Exception {
testTypes("/** @enum */ var A = {B: 1, C: 2}; " +
"/** @return {number} */ function f() { return A; }",
"inconsistent return type\n" +
"found : enum{A}\n" +
"required: number");
}
public void testEnum30() throws Exception {
testTypes("/** @enum */ var A = {B: 1, C: 2}; " +
"/** @return {number} */ function f() { return A.B; }");
}
public void testEnum31() throws Exception {
testTypes("/** @enum */ var A = {B: 1, C: 2}; " +
"/** @return {A} */ function f() { return A; }",
"inconsistent return type\n" +
"found : enum{A}\n" +
"required: A.<number>");
}
public void testEnum32() throws Exception {
testTypes("/** @enum */ var A = {B: 1, C: 2}; " +
"/** @return {A} */ function f() { return A.B; }");
}
public void testEnum34() throws Exception {
testTypes("/** @enum */ var A = {B: 1, C: 2}; " +
"/** @param {number} x */ function f(x) { return x == A.B; }");
}
public void testEnum35() throws Exception {
testTypes("var a = a || {}; /** @enum */ a.b = {C: 1, D: 2};" +
"/** @return {a.b} */ function f() { return a.b.C; }");
}
public void testEnum36() throws Exception {
testTypes("var a = a || {}; /** @enum */ a.b = {C: 1, D: 2};" +
"/** @return {!a.b} */ function f() { return 1; }",
"inconsistent return type\n" +
"found : number\n" +
"required: a.b.<number>");
}
public void testEnum37() throws Exception {
testTypes(
"var goog = goog || {};" +
"/** @enum {number} */ goog.a = {};" +
"/** @enum */ var b = goog.a;");
}
public void testEnum38() throws Exception {
testTypes(
"/** @enum {MyEnum} */ var MyEnum = {};" +
"/** @param {MyEnum} x */ function f(x) {}",
"Parse error. Cycle detected in inheritance chain " +
"of type MyEnum");
}
public void testEnum39() throws Exception {
testTypes(
"/** @enum {Number} */ var MyEnum = {FOO: new Number(1)};" +
"/** @param {MyEnum} x \n * @return {number} */" +
"function f(x) { return x == MyEnum.FOO && MyEnum.FOO == x; }",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testEnum40() throws Exception {
testTypes(
"/** @enum {Number} */ var MyEnum = {FOO: new Number(1)};" +
"/** @param {number} x \n * @return {number} */" +
"function f(x) { return x == MyEnum.FOO && MyEnum.FOO == x; }",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testEnum41() throws Exception {
testTypes(
"/** @enum {number} */ var MyEnum = {/** @const */ FOO: 1};" +
"/** @return {string} */" +
"function f() { return MyEnum.FOO; }",
"inconsistent return type\n" +
"found : MyEnum.<number>\n" +
"required: string");
}
public void testEnum42() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"/** @enum {Object} */ var MyEnum = {FOO: {newProperty: 1, b: 2}};" +
"f(MyEnum.FOO.newProperty);");
}
public void testAliasedEnum1() throws Exception {
testTypes(
"/** @enum */ var YourEnum = {FOO: 3};" +
"/** @enum */ var MyEnum = YourEnum;" +
"/** @param {MyEnum} x */ function f(x) {} f(MyEnum.FOO);");
}
public void testAliasedEnum2() throws Exception {
testTypes(
"/** @enum */ var YourEnum = {FOO: 3};" +
"/** @enum */ var MyEnum = YourEnum;" +
"/** @param {YourEnum} x */ function f(x) {} f(MyEnum.FOO);");
}
public void testAliasedEnum3() throws Exception {
testTypes(
"/** @enum */ var YourEnum = {FOO: 3};" +
"/** @enum */ var MyEnum = YourEnum;" +
"/** @param {MyEnum} x */ function f(x) {} f(YourEnum.FOO);");
}
public void testAliasedEnum4() throws Exception {
testTypes(
"/** @enum */ var YourEnum = {FOO: 3};" +
"/** @enum */ var MyEnum = YourEnum;" +
"/** @param {YourEnum} x */ function f(x) {} f(YourEnum.FOO);");
}
public void testAliasedEnum5() throws Exception {
testTypes(
"/** @enum */ var YourEnum = {FOO: 3};" +
"/** @enum */ var MyEnum = YourEnum;" +
"/** @param {string} x */ function f(x) {} f(MyEnum.FOO);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : YourEnum.<number>\n" +
"required: string");
}
public void testBackwardsEnumUse1() throws Exception {
testTypes(
"/** @return {string} */ function f() { return MyEnum.FOO; }" +
"/** @enum {string} */ var MyEnum = {FOO: 'x'};");
}
public void testBackwardsEnumUse2() throws Exception {
testTypes(
"/** @return {number} */ function f() { return MyEnum.FOO; }" +
"/** @enum {string} */ var MyEnum = {FOO: 'x'};",
"inconsistent return type\n" +
"found : MyEnum.<string>\n" +
"required: number");
}
public void testBackwardsEnumUse3() throws Exception {
testTypes(
"/** @return {string} */ function f() { return MyEnum.FOO; }" +
"/** @enum {string} */ var YourEnum = {FOO: 'x'};" +
"/** @enum {string} */ var MyEnum = YourEnum;");
}
public void testBackwardsEnumUse4() throws Exception {
testTypes(
"/** @return {number} */ function f() { return MyEnum.FOO; }" +
"/** @enum {string} */ var YourEnum = {FOO: 'x'};" +
"/** @enum {string} */ var MyEnum = YourEnum;",
"inconsistent return type\n" +
"found : YourEnum.<string>\n" +
"required: number");
}
public void testBackwardsEnumUse5() throws Exception {
testTypes(
"/** @return {string} */ function f() { return MyEnum.BAR; }" +
"/** @enum {string} */ var YourEnum = {FOO: 'x'};" +
"/** @enum {string} */ var MyEnum = YourEnum;",
"element BAR does not exist on this enum");
}
public void testBackwardsTypedefUse2() throws Exception {
testTypes(
"/** @this {MyTypedef} */ function f() {}" +
"/** @typedef {!(Date|Array)} */ var MyTypedef;");
}
public void testBackwardsTypedefUse4() throws Exception {
testTypes(
"/** @return {MyTypedef} */ function f() { return null; }" +
"/** @typedef {string} */ var MyTypedef;",
"inconsistent return type\n" +
"found : null\n" +
"required: string");
}
public void testBackwardsTypedefUse6() throws Exception {
testTypes(
"/** @return {goog.MyTypedef} */ function f() { return null; }" +
"var goog = {};" +
"/** @typedef {string} */ goog.MyTypedef;",
"inconsistent return type\n" +
"found : null\n" +
"required: string");
}
public void testBackwardsTypedefUse7() throws Exception {
testTypes(
"/** @return {goog.MyTypedef} */ function f() { return null; }" +
"var goog = {};" +
"/** @typedef {Object} */ goog.MyTypedef;");
}
public void testBackwardsTypedefUse8() throws Exception {
// Technically, this isn't quite right, because the JS runtime
// will coerce null -> the global object. But we'll punt on that for now.
testTypes(
"/** @param {!Array} x */ function g(x) {}" +
"/** @this {goog.MyTypedef} */ function f() { g(this); }" +
"var goog = {};" +
"/** @typedef {(Array|null|undefined)} */ goog.MyTypedef;");
}
public void testBackwardsTypedefUse9() throws Exception {
testTypes(
"/** @param {!Array} x */ function g(x) {}" +
"/** @this {goog.MyTypedef} */ function f() { g(this); }" +
"var goog = {};" +
"/** @typedef {(Error|null|undefined)} */ goog.MyTypedef;",
"actual parameter 1 of g does not match formal parameter\n" +
"found : Error\n" +
"required: Array");
}
public void testBackwardsTypedefUse10() throws Exception {
testTypes(
"/** @param {goog.MyEnum} x */ function g(x) {}" +
"var goog = {};" +
"/** @enum {goog.MyTypedef} */ goog.MyEnum = {FOO: 1};" +
"/** @typedef {number} */ goog.MyTypedef;" +
"g(1);",
"actual parameter 1 of g does not match formal parameter\n" +
"found : number\n" +
"required: goog.MyEnum.<number>");
}
public void testBackwardsConstructor1() throws Exception {
testTypes(
"function f() { (new Foo(true)); }" +
"/** \n * @constructor \n * @param {number} x */" +
"var Foo = function(x) {};",
"actual parameter 1 of Foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testBackwardsConstructor2() throws Exception {
testTypes(
"function f() { (new Foo(true)); }" +
"/** \n * @constructor \n * @param {number} x */" +
"var YourFoo = function(x) {};" +
"/** \n * @constructor \n * @param {number} x */" +
"var Foo = YourFoo;",
"actual parameter 1 of Foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testMinimalConstructorAnnotation() throws Exception {
testTypes("/** @constructor */function Foo(){}");
}
public void testGoodExtends1() throws Exception {
// A minimal @extends example
testTypes("/** @constructor */function base() {}\n" +
"/** @constructor\n * @extends {base} */function derived() {}\n");
}
public void testGoodExtends2() throws Exception {
testTypes("/** @constructor\n * @extends base */function derived() {}\n" +
"/** @constructor */function base() {}\n");
}
public void testGoodExtends3() throws Exception {
testTypes("/** @constructor\n * @extends {Object} */function base() {}\n" +
"/** @constructor\n * @extends {base} */function derived() {}\n");
}
public void testGoodExtends4() throws Exception {
// Ensure that @extends actually sets the base type of a constructor
// correctly. Because this isn't part of the human-readable Function
// definition, we need to crawl the prototype chain (eww).
Node n = parseAndTypeCheck(
"var goog = {};\n" +
"/** @constructor */goog.Base = function(){};\n" +
"/** @constructor\n" +
" * @extends {goog.Base} */goog.Derived = function(){};\n");
Node subTypeName = n.getLastChild().getLastChild().getFirstChild();
assertEquals("goog.Derived", subTypeName.getQualifiedName());
FunctionType subCtorType =
(FunctionType) subTypeName.getNext().getJSType();
assertEquals("goog.Derived", subCtorType.getInstanceType().toString());
JSType superType = subCtorType.getPrototype().getImplicitPrototype();
assertEquals("goog.Base", superType.toString());
}
public void testGoodExtends5() throws Exception {
// we allow for the extends annotation to be placed first
testTypes("/** @constructor */function base() {}\n" +
"/** @extends {base}\n * @constructor */function derived() {}\n");
}
public void testGoodExtends6() throws Exception {
testFunctionType(
CLOSURE_DEFS +
"/** @constructor */function base() {}\n" +
"/** @return {number} */ " +
" base.prototype.foo = function() { return 1; };\n" +
"/** @extends {base}\n * @constructor */function derived() {}\n" +
"goog.inherits(derived, base);",
"derived.superClass_.foo",
"function (this:base): number");
}
public void testGoodExtends7() throws Exception {
testFunctionType(
"Function.prototype.inherits = function(x) {};" +
"/** @constructor */function base() {}\n" +
"/** @extends {base}\n * @constructor */function derived() {}\n" +
"derived.inherits(base);",
"(new derived).constructor",
"function (new:derived, ...[?]): ?");
}
public void testGoodExtends8() throws Exception {
testTypes("/** @constructor \n @extends {Base} */ function Sub() {}" +
"/** @return {number} */ function f() { return (new Sub()).foo; }" +
"/** @constructor */ function Base() {}" +
"/** @type {boolean} */ Base.prototype.foo = true;",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testGoodExtends9() throws Exception {
testTypes(
"/** @constructor */ function Super() {}" +
"Super.prototype.foo = function() {};" +
"/** @constructor \n * @extends {Super} */ function Sub() {}" +
"Sub.prototype = new Super();" +
"/** @override */ Sub.prototype.foo = function() {};");
}
public void testGoodExtends10() throws Exception {
testTypes(
"/** @constructor */ function Super() {}" +
"/** @constructor \n * @extends {Super} */ function Sub() {}" +
"Sub.prototype = new Super();" +
"/** @return {Super} */ function foo() { return new Sub(); }");
}
public void testGoodExtends11() throws Exception {
testTypes(
"/** @constructor */ function Super() {}" +
"/** @param {boolean} x */ Super.prototype.foo = function(x) {};" +
"/** @constructor \n * @extends {Super} */ function Sub() {}" +
"Sub.prototype = new Super();" +
"(new Sub()).foo(0);",
"actual parameter 1 of Super.prototype.foo " +
"does not match formal parameter\n" +
"found : number\n" +
"required: boolean");
}
public void testGoodExtends12() throws Exception {
testTypes(
"/** @constructor \n * @extends {Super} */ function Sub() {}" +
"/** @constructor \n * @extends {Sub} */ function Sub2() {}" +
"/** @constructor */ function Super() {}" +
"/** @param {Super} x */ function foo(x) {}" +
"foo(new Sub2());");
}
public void testGoodExtends13() throws Exception {
testTypes(
"/** @constructor \n * @extends {B} */ function C() {}" +
"/** @constructor \n * @extends {D} */ function E() {}" +
"/** @constructor \n * @extends {C} */ function D() {}" +
"/** @constructor \n * @extends {A} */ function B() {}" +
"/** @constructor */ function A() {}" +
"/** @param {number} x */ function f(x) {} f(new E());",
"actual parameter 1 of f does not match formal parameter\n" +
"found : E\n" +
"required: number");
}
public void testGoodExtends14() throws Exception {
testTypes(
CLOSURE_DEFS +
"/** @param {Function} f */ function g(f) {" +
" /** @constructor */ function NewType() {};" +
" goog.inherits(NewType, f);" +
" (new NewType());" +
"}");
}
public void testGoodExtends15() throws Exception {
testTypes(
CLOSURE_DEFS +
"/** @constructor */ function OldType() {}" +
"/** @param {?function(new:OldType)} f */ function g(f) {" +
" /**\n" +
" * @constructor\n" +
" * @extends {OldType}\n" +
" */\n" +
" function NewType() {};" +
" goog.inherits(NewType, f);" +
" NewType.prototype.method = function() {" +
" NewType.superClass_.foo.call(this);" +
" };" +
"}",
"Property foo never defined on OldType.prototype");
}
public void testGoodExtends16() throws Exception {
testTypes(
CLOSURE_DEFS +
"/** @param {Function} f */ function g(f) {" +
" /** @constructor */ function NewType() {};" +
" goog.inherits(f, NewType);" +
" (new NewType());" +
"}");
}
public void testGoodExtends17() throws Exception {
testFunctionType(
"Function.prototype.inherits = function(x) {};" +
"/** @constructor */function base() {}\n" +
"/** @param {number} x */ base.prototype.bar = function(x) {};\n" +
"/** @extends {base}\n * @constructor */function derived() {}\n" +
"derived.inherits(base);",
"(new derived).constructor.prototype.bar",
"function (this:base, number): undefined");
}
public void testBadExtends1() throws Exception {
testTypes("/** @constructor */function base() {}\n" +
"/** @constructor\n * @extends {not_base} */function derived() {}\n",
"Bad type annotation. Unknown type not_base");
}
public void testBadExtends2() throws Exception {
testTypes("/** @constructor */function base() {\n" +
"/** @type {!Number}*/\n" +
"this.baseMember = new Number(4);\n" +
"}\n" +
"/** @constructor\n" +
" * @extends {base} */function derived() {}\n" +
"/** @param {!String} x*/\n" +
"function foo(x){ }\n" +
"/** @type {!derived}*/var y;\n" +
"foo(y.baseMember);\n",
"actual parameter 1 of foo does not match formal parameter\n" +
"found : Number\n" +
"required: String");
}
public void testBadExtends3() throws Exception {
testTypes("/** @extends {Object} */function base() {}",
"@extends used without @constructor or @interface for base");
}
public void testBadExtends4() throws Exception {
// If there's a subclass of a class with a bad extends,
// we only want to warn about the first one.
testTypes(
"/** @constructor \n * @extends {bad} */ function Sub() {}" +
"/** @constructor \n * @extends {Sub} */ function Sub2() {}" +
"/** @param {Sub} x */ function foo(x) {}" +
"foo(new Sub2());",
"Bad type annotation. Unknown type bad");
}
public void testLateExtends() throws Exception {
testTypes(
CLOSURE_DEFS +
"/** @constructor */ function Foo() {}\n" +
"Foo.prototype.foo = function() {};\n" +
"/** @constructor */function Bar() {}\n" +
"goog.inherits(Foo, Bar);\n",
"Missing @extends tag on type Foo");
}
public void testSuperclassMatch() throws Exception {
compiler.getOptions().setCodingConvention(new GoogleCodingConvention());
testTypes("/** @constructor */ var Foo = function() {};\n" +
"/** @constructor \n @extends Foo */ var Bar = function() {};\n" +
"Bar.inherits = function(x){};" +
"Bar.inherits(Foo);\n");
}
public void testSuperclassMatchWithMixin() throws Exception {
compiler.getOptions().setCodingConvention(new GoogleCodingConvention());
testTypes("/** @constructor */ var Foo = function() {};\n" +
"/** @constructor */ var Baz = function() {};\n" +
"/** @constructor \n @extends Foo */ var Bar = function() {};\n" +
"Bar.inherits = function(x){};" +
"Bar.mixin = function(y){};" +
"Bar.inherits(Foo);\n" +
"Bar.mixin(Baz);\n");
}
public void testSuperclassMismatch1() throws Exception {
compiler.getOptions().setCodingConvention(new GoogleCodingConvention());
testTypes("/** @constructor */ var Foo = function() {};\n" +
"/** @constructor \n @extends Object */ var Bar = function() {};\n" +
"Bar.inherits = function(x){};" +
"Bar.inherits(Foo);\n",
"Missing @extends tag on type Bar");
}
public void testSuperclassMismatch2() throws Exception {
compiler.getOptions().setCodingConvention(new GoogleCodingConvention());
testTypes("/** @constructor */ var Foo = function(){};\n" +
"/** @constructor */ var Bar = function(){};\n" +
"Bar.inherits = function(x){};" +
"Bar.inherits(Foo);",
"Missing @extends tag on type Bar");
}
public void testSuperClassDefinedAfterSubClass1() throws Exception {
testTypes(
"/** @constructor \n * @extends {Base} */ function A() {}" +
"/** @constructor \n * @extends {Base} */ function B() {}" +
"/** @constructor */ function Base() {}" +
"/** @param {A|B} x \n * @return {B|A} */ " +
"function foo(x) { return x; }");
}
public void testSuperClassDefinedAfterSubClass2() throws Exception {
testTypes(
"/** @constructor \n * @extends {Base} */ function A() {}" +
"/** @constructor \n * @extends {Base} */ function B() {}" +
"/** @param {A|B} x \n * @return {B|A} */ " +
"function foo(x) { return x; }" +
"/** @constructor */ function Base() {}");
}
public void testDirectPrototypeAssignment1() throws Exception {
testTypes(
"/** @constructor */ function Base() {}" +
"Base.prototype.foo = 3;" +
"/** @constructor \n * @extends {Base} */ function A() {}" +
"A.prototype = new Base();" +
"/** @return {string} */ function foo() { return (new A).foo; }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testDirectPrototypeAssignment2() throws Exception {
// This ensures that we don't attach property 'foo' onto the Base
// instance object.
testTypes(
"/** @constructor */ function Base() {}" +
"/** @constructor \n * @extends {Base} */ function A() {}" +
"A.prototype = new Base();" +
"A.prototype.foo = 3;" +
"/** @return {string} */ function foo() { return (new Base).foo; }");
}
public void testDirectPrototypeAssignment3() throws Exception {
// This verifies that the compiler doesn't crash if the user
// overwrites the prototype of a global variable in a local scope.
testTypes(
"/** @constructor */ var MainWidgetCreator = function() {};" +
"/** @param {Function} ctor */" +
"function createMainWidget(ctor) {" +
" /** @constructor */ function tempCtor() {};" +
" tempCtor.prototype = ctor.prototype;" +
" MainWidgetCreator.superClass_ = ctor.prototype;" +
" MainWidgetCreator.prototype = new tempCtor();" +
"}");
}
public void testGoodImplements1() throws Exception {
testTypes("/** @interface */function Disposable() {}\n" +
"/** @implements {Disposable}\n * @constructor */function f() {}");
}
public void testGoodImplements2() throws Exception {
testTypes("/** @interface */function Base1() {}\n" +
"/** @interface */function Base2() {}\n" +
"/** @constructor\n" +
" * @implements {Base1}\n" +
" * @implements {Base2}\n" +
" */ function derived() {}");
}
public void testGoodImplements3() throws Exception {
testTypes("/** @interface */function Disposable() {}\n" +
"/** @constructor \n @implements {Disposable} */function f() {}");
}
public void testGoodImplements4() throws Exception {
testTypes("var goog = {};" +
"/** @type {!Function} */" +
"goog.abstractMethod = function() {};" +
"/** @interface */\n" +
"goog.Disposable = goog.abstractMethod;" +
"goog.Disposable.prototype.dispose = goog.abstractMethod;" +
"/** @implements {goog.Disposable}\n * @constructor */" +
"goog.SubDisposable = function() {};" +
"/** @inheritDoc */ " +
"goog.SubDisposable.prototype.dispose = function() {};");
}
public void testGoodImplements5() throws Exception {
testTypes(
"/** @interface */\n" +
"goog.Disposable = function() {};" +
"/** @type {Function} */" +
"goog.Disposable.prototype.dispose = function() {};" +
"/** @implements {goog.Disposable}\n * @constructor */" +
"goog.SubDisposable = function() {};" +
"/** @param {number} key \n @override */ " +
"goog.SubDisposable.prototype.dispose = function(key) {};");
}
public void testGoodImplements6() throws Exception {
testTypes(
"var myNullFunction = function() {};" +
"/** @interface */\n" +
"goog.Disposable = function() {};" +
"/** @return {number} */" +
"goog.Disposable.prototype.dispose = myNullFunction;" +
"/** @implements {goog.Disposable}\n * @constructor */" +
"goog.SubDisposable = function() {};" +
"/** @return {number} \n @override */ " +
"goog.SubDisposable.prototype.dispose = function() { return 0; };");
}
public void testGoodImplements7() throws Exception {
testTypes(
"var myNullFunction = function() {};" +
"/** @interface */\n" +
"goog.Disposable = function() {};" +
"/** @return {number} */" +
"goog.Disposable.prototype.dispose = function() {};" +
"/** @implements {goog.Disposable}\n * @constructor */" +
"goog.SubDisposable = function() {};" +
"/** @return {number} \n @override */ " +
"goog.SubDisposable.prototype.dispose = function() { return 0; };");
}
public void testBadImplements1() throws Exception {
testTypes("/** @interface */function Base1() {}\n" +
"/** @interface */function Base2() {}\n" +
"/** @constructor\n" +
" * @implements {nonExistent}\n" +
" * @implements {Base2}\n" +
" */ function derived() {}",
"Bad type annotation. Unknown type nonExistent");
}
public void testBadImplements2() throws Exception {
testTypes("/** @interface */function Disposable() {}\n" +
"/** @implements {Disposable}\n */function f() {}",
"@implements used without @constructor for f");
}
public void testBadImplements3() throws Exception {
testTypes(
"var goog = {};" +
"/** @type {!Function} */ goog.abstractMethod = function(){};" +
"/** @interface */ var Disposable = goog.abstractMethod;" +
"Disposable.prototype.method = goog.abstractMethod;" +
"/** @implements {Disposable}\n * @constructor */function f() {}",
"property method on interface Disposable is not implemented by type f");
}
public void testBadImplements4() throws Exception {
testTypes("/** @interface */function Disposable() {}\n" +
"/** @implements {Disposable}\n * @interface */function f() {}",
"f cannot implement this type; an interface can only extend, " +
"but not implement interfaces");
}
public void testBadImplements5() throws Exception {
testTypes("/** @interface */function Disposable() {}\n" +
"/** @type {number} */ Disposable.prototype.bar = function() {};",
"assignment to property bar of Disposable.prototype\n" +
"found : function (): undefined\n" +
"required: number");
}
public void testBadImplements6() throws Exception {
testClosureTypesMultipleWarnings(
"/** @interface */function Disposable() {}\n" +
"/** @type {function()} */ Disposable.prototype.bar = 3;",
Lists.newArrayList(
"assignment to property bar of Disposable.prototype\n" +
"found : number\n" +
"required: function (): ?",
"interface members can only be empty property declarations, " +
"empty functions, or goog.abstractMethod"));
}
public void testInterfaceExtends() throws Exception {
testTypes("/** @interface */function A() {}\n" +
"/** @interface \n * @extends {A} */function B() {}\n" +
"/** @constructor\n" +
" * @implements {B}\n" +
" */ function derived() {}");
}
public void testBadInterfaceExtends1() throws Exception {
testTypes("/** @interface \n * @extends {nonExistent} */function A() {}",
"Bad type annotation. Unknown type nonExistent");
}
public void testBadInterfaceExtends2() throws Exception {
testTypes("/** @constructor */function A() {}\n" +
"/** @interface \n * @extends {A} */function B() {}",
"B cannot extend this type; interfaces can only extend interfaces");
}
public void testBadInterfaceExtends3() throws Exception {
testTypes("/** @interface */function A() {}\n" +
"/** @constructor \n * @extends {A} */function B() {}",
"B cannot extend this type; constructors can only extend constructors");
}
public void testBadInterfaceExtends4() throws Exception {
// TODO(user): This should be detected as an error. Even if we enforce
// that A cannot be used in the assignment, we should still detect the
// inheritance chain as invalid.
testTypes("/** @interface */function A() {}\n" +
"/** @constructor */function B() {}\n" +
"B.prototype = A;");
}
public void testBadInterfaceExtends5() throws Exception {
// TODO(user): This should be detected as an error. Even if we enforce
// that A cannot be used in the assignment, we should still detect the
// inheritance chain as invalid.
testTypes("/** @constructor */function A() {}\n" +
"/** @interface */function B() {}\n" +
"B.prototype = A;");
}
public void testBadImplementsAConstructor() throws Exception {
testTypes("/** @constructor */function A() {}\n" +
"/** @constructor \n * @implements {A} */function B() {}",
"can only implement interfaces");
}
public void testBadImplementsNonInterfaceType() throws Exception {
testTypes("/** @constructor \n * @implements {Boolean} */function B() {}",
"can only implement interfaces");
}
public void testBadImplementsNonObjectType() throws Exception {
testTypes("/** @constructor \n * @implements {string} */function S() {}",
"can only implement interfaces");
}
public void testInterfaceAssignment1() throws Exception {
testTypes("/** @interface */var I = function() {};\n" +
"/** @constructor\n@implements {I} */var T = function() {};\n" +
"var t = new T();\n" +
"/** @type {!I} */var i = t;");
}
public void testInterfaceAssignment2() throws Exception {
testTypes("/** @interface */var I = function() {};\n" +
"/** @constructor */var T = function() {};\n" +
"var t = new T();\n" +
"/** @type {!I} */var i = t;",
"initializing variable\n" +
"found : T\n" +
"required: I");
}
public void testInterfaceAssignment3() throws Exception {
testTypes("/** @interface */var I = function() {};\n" +
"/** @constructor\n@implements {I} */var T = function() {};\n" +
"var t = new T();\n" +
"/** @type {I|number} */var i = t;");
}
public void testInterfaceAssignment4() throws Exception {
testTypes("/** @interface */var I1 = function() {};\n" +
"/** @interface */var I2 = function() {};\n" +
"/** @constructor\n@implements {I1} */var T = function() {};\n" +
"var t = new T();\n" +
"/** @type {I1|I2} */var i = t;");
}
public void testInterfaceAssignment5() throws Exception {
testTypes("/** @interface */var I1 = function() {};\n" +
"/** @interface */var I2 = function() {};\n" +
"/** @constructor\n@implements {I1}\n@implements {I2}*/" +
"var T = function() {};\n" +
"var t = new T();\n" +
"/** @type {I1} */var i1 = t;\n" +
"/** @type {I2} */var i2 = t;\n");
}
public void testInterfaceAssignment6() throws Exception {
testTypes("/** @interface */var I1 = function() {};\n" +
"/** @interface */var I2 = function() {};\n" +
"/** @constructor\n@implements {I1} */var T = function() {};\n" +
"/** @type {!I1} */var i1 = new T();\n" +
"/** @type {!I2} */var i2 = i1;\n",
"initializing variable\n" +
"found : I1\n" +
"required: I2");
}
public void testInterfaceAssignment7() throws Exception {
testTypes("/** @interface */var I1 = function() {};\n" +
"/** @interface\n@extends {I1}*/var I2 = function() {};\n" +
"/** @constructor\n@implements {I2}*/var T = function() {};\n" +
"var t = new T();\n" +
"/** @type {I1} */var i1 = t;\n" +
"/** @type {I2} */var i2 = t;\n" +
"i1 = i2;\n");
}
public void testInterfaceAssignment8() throws Exception {
testTypes("/** @interface */var I = function() {};\n" +
"/** @type {I} */var i;\n" +
"/** @type {Object} */var o = i;\n" +
"new Object().prototype = i.prototype;");
}
public void testInterfaceAssignment9() throws Exception {
testTypes("/** @interface */var I = function() {};\n" +
"/** @return {I?} */function f() { return null; }\n" +
"/** @type {!I} */var i = f();\n",
"initializing variable\n" +
"found : (I|null)\n" +
"required: I");
}
public void testInterfaceAssignment10() throws Exception {
testTypes("/** @interface */var I1 = function() {};\n" +
"/** @interface */var I2 = function() {};\n" +
"/** @constructor\n@implements {I2} */var T = function() {};\n" +
"/** @return {!I1|!I2} */function f() { return new T(); }\n" +
"/** @type {!I1} */var i1 = f();\n",
"initializing variable\n" +
"found : (I1|I2)\n" +
"required: I1");
}
public void testInterfaceAssignment11() throws Exception {
testTypes("/** @interface */var I1 = function() {};\n" +
"/** @interface */var I2 = function() {};\n" +
"/** @constructor */var T = function() {};\n" +
"/** @return {!I1|!I2|!T} */function f() { return new T(); }\n" +
"/** @type {!I1} */var i1 = f();\n",
"initializing variable\n" +
"found : (I1|I2|T)\n" +
"required: I1");
}
public void testInterfaceAssignment12() throws Exception {
testTypes("/** @interface */var I = function() {};\n" +
"/** @constructor\n@implements{I}*/var T1 = function() {};\n" +
"/** @constructor\n@extends {T1}*/var T2 = function() {};\n" +
"/** @return {I} */function f() { return new T2(); }");
}
public void testInterfaceAssignment13() throws Exception {
testTypes("/** @interface */var I = function() {};\n" +
"/** @constructor\n@implements {I}*/var T = function() {};\n" +
"/** @constructor */function Super() {};\n" +
"/** @return {I} */Super.prototype.foo = " +
"function() { return new T(); };\n" +
"/** @constructor\n@extends {Super} */function Sub() {}\n" +
"/** @override\n@return {T} */Sub.prototype.foo = " +
"function() { return new T(); };\n");
}
public void testGetprop1() throws Exception {
testTypes("/** @return {void}*/function foo(){foo().bar;}",
"No properties on this expression\n" +
"found : undefined\n" +
"required: Object");
}
public void testGetprop2() throws Exception {
testTypes("var x = null; x.alert();",
"No properties on this expression\n" +
"found : null\n" +
"required: Object");
}
public void testGetprop3() throws Exception {
testTypes(
"/** @constructor */ " +
"function Foo() { /** @type {?Object} */ this.x = null; }" +
"Foo.prototype.initX = function() { this.x = {foo: 1}; };" +
"Foo.prototype.bar = function() {" +
" if (this.x == null) { this.initX(); alert(this.x.foo); }" +
"};");
}
public void testGetprop4() throws Exception {
testTypes("var x = null; x.prop = 3;",
"No properties on this expression\n" +
"found : null\n" +
"required: Object");
}
public void testSetprop1() throws Exception {
// Create property on struct in the constructor
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */\n" +
"function Foo() { this.x = 123; }");
}
public void testSetprop2() throws Exception {
// Create property on struct outside the constructor
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */\n" +
"function Foo() {}\n" +
"(new Foo()).x = 123;",
"Cannot add a property to a struct instance " +
"after it is constructed.");
}
public void testSetprop3() throws Exception {
// Create property on struct outside the constructor
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */\n" +
"function Foo() {}\n" +
"(function() { (new Foo()).x = 123; })();",
"Cannot add a property to a struct instance " +
"after it is constructed.");
}
public void testSetprop4() throws Exception {
// Assign to existing property of struct outside the constructor
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */\n" +
"function Foo() { this.x = 123; }\n" +
"(new Foo()).x = \"asdf\";");
}
public void testSetprop5() throws Exception {
// Create a property on union that includes a struct
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */\n" +
"function Foo() {}\n" +
"(true ? new Foo() : {}).x = 123;",
"Cannot add a property to a struct instance " +
"after it is constructed.");
}
public void testSetprop6() throws Exception {
// Create property on struct in another constructor
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */\n" +
"function Foo() {}\n" +
"/**\n" +
" * @constructor\n" +
" * @param{Foo} f\n" +
" */\n" +
"function Bar(f) { f.x = 123; }",
"Cannot add a property to a struct instance " +
"after it is constructed.");
}
public void testSetprop7() throws Exception {
//Bug b/c we require THIS when creating properties on structs for simplicity
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */\n" +
"function Foo() {\n" +
" var t = this;\n" +
" t.x = 123;\n" +
"}",
"Cannot add a property to a struct instance " +
"after it is constructed.");
}
public void testSetprop8() throws Exception {
// Create property on struct using DEC
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */\n" +
"function Foo() {}\n" +
"(new Foo()).x--;",
new String[] {
"Property x never defined on Foo",
"Cannot add a property to a struct instance " +
"after it is constructed."
});
}
public void testSetprop9() throws Exception {
// Create property on struct using ASSIGN_ADD
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */\n" +
"function Foo() {}\n" +
"(new Foo()).x += 123;",
new String[] {
"Property x never defined on Foo",
"Cannot add a property to a struct instance " +
"after it is constructed."
});
}
public void testSetprop10() throws Exception {
// Create property on object literal that is a struct
testTypes("/** \n" +
" * @constructor \n" +
" * @struct \n" +
" */ \n" +
"function Square(side) { \n" +
" this.side = side; \n" +
"} \n" +
"Square.prototype = /** @struct */ {\n" +
" area: function() { return this.side * this.side; }\n" +
"};\n" +
"Square.prototype.id = function(x) { return x; };\n",
"Cannot add a property to a struct instance " +
"after it is constructed.");
}
public void testSetprop11() throws Exception {
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */\n" +
"function Foo() {}\n" +
"function Bar() {}\n" +
"Bar.prototype = new Foo();\n" +
"Bar.prototype.someprop = 123;\n",
"Cannot add a property to a struct instance " +
"after it is constructed.");
}
public void testGetpropDict1() throws Exception {
testTypes("/**\n" +
" * @constructor\n" +
" * @dict\n" +
" */" +
"function Dict1(){ this['prop'] = 123; }" +
"/** @param{Dict1} x */" +
"function takesDict(x) { return x.prop; }",
"Cannot do '.' access on a dict");
}
public void testGetpropDict2() throws Exception {
testTypes("/**\n" +
" * @constructor\n" +
" * @dict\n" +
" */" +
"function Dict1(){ this['prop'] = 123; }" +
"/**\n" +
" * @constructor\n" +
" * @extends {Dict1}\n" +
" */" +
"function Dict1kid(){ this['prop'] = 123; }" +
"/** @param{Dict1kid} x */" +
"function takesDict(x) { return x.prop; }",
"Cannot do '.' access on a dict");
}
public void testGetpropDict3() throws Exception {
testTypes("/**\n" +
" * @constructor\n" +
" * @dict\n" +
" */" +
"function Dict1() { this['prop'] = 123; }" +
"/** @constructor */" +
"function NonDict() { this.prop = 321; }" +
"/** @param{(NonDict|Dict1)} x */" +
"function takesDict(x) { return x.prop; }",
"Cannot do '.' access on a dict");
}
public void testGetpropDict4() throws Exception {
testTypes("/**\n" +
" * @constructor\n" +
" * @dict\n" +
" */" +
"function Dict1() { this['prop'] = 123; }" +
"/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */" +
"function Struct1() { this.prop = 123; }" +
"/** @param{(Struct1|Dict1)} x */" +
"function takesNothing(x) { return x.prop; }",
"Cannot do '.' access on a dict");
}
public void testGetpropDict5() throws Exception {
testTypes("/**\n" +
" * @constructor\n" +
" * @dict\n" +
" */" +
"function Dict1(){ this.prop = 123; }",
"Cannot do '.' access on a dict");
}
public void testGetpropDict6() throws Exception {
testTypes("/**\n" +
" * @constructor\n" +
" * @dict\n" +
" */\n" +
"function Foo() {}\n" +
"function Bar() {}\n" +
"Bar.prototype = new Foo();\n" +
"Bar.prototype.someprop = 123;\n",
"Cannot do '.' access on a dict");
}
public void testGetpropDict7() throws Exception {
testTypes("(/** @dict */ {x: 123}).x = 321;",
"Cannot do '.' access on a dict");
}
public void testGetelemStruct1() throws Exception {
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */" +
"function Struct1(){ this.prop = 123; }" +
"/** @param{Struct1} x */" +
"function takesStruct(x) {" +
" var z = x;" +
" return z['prop'];" +
"}",
"Cannot do '[]' access on a struct");
}
public void testGetelemStruct2() throws Exception {
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */" +
"function Struct1(){ this.prop = 123; }" +
"/**\n" +
" * @constructor\n" +
" * @extends {Struct1}" +
" */" +
"function Struct1kid(){ this.prop = 123; }" +
"/** @param{Struct1kid} x */" +
"function takesStruct2(x) { return x['prop']; }",
"Cannot do '[]' access on a struct");
}
public void testGetelemStruct3() throws Exception {
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */" +
"function Struct1(){ this.prop = 123; }" +
"/**\n" +
" * @constructor\n" +
" * @extends {Struct1}\n" +
" */" +
"function Struct1kid(){ this.prop = 123; }" +
"var x = (new Struct1kid())['prop'];",
"Cannot do '[]' access on a struct");
}
public void testGetelemStruct4() throws Exception {
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */" +
"function Struct1() { this.prop = 123; }" +
"/** @constructor */" +
"function NonStruct() { this.prop = 321; }" +
"/** @param{(NonStruct|Struct1)} x */" +
"function takesStruct(x) { return x['prop']; }",
"Cannot do '[]' access on a struct");
}
public void testGetelemStruct5() throws Exception {
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */" +
"function Struct1() { this.prop = 123; }" +
"/**\n" +
" * @constructor\n" +
" * @dict\n" +
" */" +
"function Dict1() { this['prop'] = 123; }" +
"/** @param{(Struct1|Dict1)} x */" +
"function takesNothing(x) { return x['prop']; }",
"Cannot do '[]' access on a struct");
}
public void testGetelemStruct6() throws Exception {
// By casting Bar to Foo, the illegal bracket access is not detected
testTypes("/** @interface */ function Foo(){}\n" +
"/**\n" +
" * @constructor\n" +
" * @struct\n" +
" * @implements {Foo}\n" +
" */" +
"function Bar(){ this.x = 123; }\n" +
"var z = /** @type {Foo} */(new Bar)['x'];");
}
public void testGetelemStruct7() throws Exception {
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */\n" +
"function Foo() {}\n" +
"function Bar() {}\n" +
"Bar.prototype = new Foo();\n" +
"Bar.prototype['someprop'] = 123;\n",
"Cannot do '[]' access on a struct");
}
public void testInOnStruct() throws Exception {
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */" +
"function Foo() {}\n" +
"if ('prop' in (new Foo())) {}",
"Cannot use the IN operator with structs");
}
public void testForinOnStruct() throws Exception {
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */" +
"function Foo() {}\n" +
"for (var prop in (new Foo())) {}",
"Cannot use the IN operator with structs");
}
public void testArrayAccess1() throws Exception {
testTypes("var a = []; var b = a['hi'];");
}
public void testArrayAccess2() throws Exception {
testTypes("var a = []; var b = a[[1,2]];",
"array access\n" +
"found : Array\n" +
"required: number");
}
public void testArrayAccess3() throws Exception {
testTypes("var bar = [];" +
"/** @return {void} */function baz(){};" +
"var foo = bar[baz()];",
"array access\n" +
"found : undefined\n" +
"required: number");
}
public void testArrayAccess4() throws Exception {
testTypes("/**@return {!Array}*/function foo(){};var bar = foo()[foo()];",
"array access\n" +
"found : Array\n" +
"required: number");
}
public void testArrayAccess6() throws Exception {
testTypes("var bar = null[1];",
"only arrays or objects can be accessed\n" +
"found : null\n" +
"required: Object");
}
public void testArrayAccess7() throws Exception {
testTypes("var bar = void 0; bar[0];",
"only arrays or objects can be accessed\n" +
"found : undefined\n" +
"required: Object");
}
public void testArrayAccess8() throws Exception {
// Verifies that we don't emit two warnings, because
// the var has been dereferenced after the first one.
testTypes("var bar = void 0; bar[0]; bar[1];",
"only arrays or objects can be accessed\n" +
"found : undefined\n" +
"required: Object");
}
public void testArrayAccess9() throws Exception {
testTypes("/** @return {?Array} */ function f() { return []; }" +
"f()[{}]",
"array access\n" +
"found : {}\n" +
"required: number");
}
public void testPropAccess() throws Exception {
testTypes("/** @param {*} x */var f = function(x) {\n" +
"var o = String(x);\n" +
"if (typeof o['a'] != 'undefined') { return o['a']; }\n" +
"return null;\n" +
"};");
}
public void testPropAccess2() throws Exception {
testTypes("var bar = void 0; bar.baz;",
"No properties on this expression\n" +
"found : undefined\n" +
"required: Object");
}
public void testPropAccess3() throws Exception {
// Verifies that we don't emit two warnings, because
// the var has been dereferenced after the first one.
testTypes("var bar = void 0; bar.baz; bar.bax;",
"No properties on this expression\n" +
"found : undefined\n" +
"required: Object");
}
public void testPropAccess4() throws Exception {
testTypes("/** @param {*} x */ function f(x) { return x['hi']; }");
}
public void testSwitchCase1() throws Exception {
testTypes("/**@type number*/var a;" +
"/**@type string*/var b;" +
"switch(a){case b:;}",
"case expression doesn't match switch\n" +
"found : string\n" +
"required: number");
}
public void testSwitchCase2() throws Exception {
testTypes("var a = null; switch (typeof a) { case 'foo': }");
}
public void testVar1() throws Exception {
TypeCheckResult p =
parseAndTypeCheckWithScope("/** @type {(string,null)} */var a = null");
assertTypeEquals(createUnionType(STRING_TYPE, NULL_TYPE),
p.scope.getVar("a").getType());
}
public void testVar2() throws Exception {
testTypes("/** @type {Function} */ var a = function(){}");
}
public void testVar3() throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope("var a = 3;");
assertTypeEquals(NUMBER_TYPE, p.scope.getVar("a").getType());
}
public void testVar4() throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope(
"var a = 3; a = 'string';");
assertTypeEquals(createUnionType(STRING_TYPE, NUMBER_TYPE),
p.scope.getVar("a").getType());
}
public void testVar5() throws Exception {
testTypes("var goog = {};" +
"/** @type string */goog.foo = 'hello';" +
"/** @type number */var a = goog.foo;",
"initializing variable\n" +
"found : string\n" +
"required: number");
}
public void testVar6() throws Exception {
testTypes(
"function f() {" +
" return function() {" +
" /** @type {!Date} */" +
" var a = 7;" +
" };" +
"}",
"initializing variable\n" +
"found : number\n" +
"required: Date");
}
public void testVar7() throws Exception {
testTypes("/** @type number */var a, b;",
"declaration of multiple variables with shared type information");
}
public void testVar8() throws Exception {
testTypes("var a, b;");
}
public void testVar9() throws Exception {
testTypes("/** @enum */var a;",
"enum initializer must be an object literal or an enum");
}
public void testVar10() throws Exception {
testTypes("/** @type !Number */var foo = 'abc';",
"initializing variable\n" +
"found : string\n" +
"required: Number");
}
public void testVar11() throws Exception {
testTypes("var /** @type !Date */foo = 'abc';",
"initializing variable\n" +
"found : string\n" +
"required: Date");
}
public void testVar12() throws Exception {
testTypes("var /** @type !Date */foo = 'abc', " +
"/** @type !RegExp */bar = 5;",
new String[] {
"initializing variable\n" +
"found : string\n" +
"required: Date",
"initializing variable\n" +
"found : number\n" +
"required: RegExp"});
}
public void testVar13() throws Exception {
// this caused an NPE
testTypes("var /** @type number */a,a;");
}
public void testVar14() throws Exception {
testTypes("/** @return {number} */ function f() { var x; return x; }",
"inconsistent return type\n" +
"found : undefined\n" +
"required: number");
}
public void testVar15() throws Exception {
testTypes("/** @return {number} */" +
"function f() { var x = x || {}; return x; }",
"inconsistent return type\n" +
"found : {}\n" +
"required: number");
}
public void testAssign1() throws Exception {
testTypes("var goog = {};" +
"/** @type number */goog.foo = 'hello';",
"assignment to property foo of goog\n" +
"found : string\n" +
"required: number");
}
public void testAssign2() throws Exception {
testTypes("var goog = {};" +
"/** @type number */goog.foo = 3;" +
"goog.foo = 'hello';",
"assignment to property foo of goog\n" +
"found : string\n" +
"required: number");
}
public void testAssign3() throws Exception {
testTypes("var goog = {};" +
"/** @type number */goog.foo = 3;" +
"goog.foo = 4;");
}
public void testAssign4() throws Exception {
testTypes("var goog = {};" +
"goog.foo = 3;" +
"goog.foo = 'hello';");
}
public void testAssignInference() throws Exception {
testTypes(
"/**" +
" * @param {Array} x" +
" * @return {number}" +
" */" +
"function f(x) {" +
" var y = null;" +
" y = x[0];" +
" if (y == null) { return 4; } else { return 6; }" +
"}");
}
public void testOr1() throws Exception {
testTypes("/** @type number */var a;" +
"/** @type number */var b;" +
"a + b || undefined;");
}
public void testOr2() throws Exception {
testTypes("/** @type number */var a;" +
"/** @type number */var b;" +
"/** @type number */var c = a + b || undefined;",
"initializing variable\n" +
"found : (number|undefined)\n" +
"required: number");
}
public void testOr3() throws Exception {
testTypes("/** @type {(number, undefined)} */var a;" +
"/** @type number */var c = a || 3;");
}
/**
* Test that type inference continues with the right side,
* when no short-circuiting is possible.
* See bugid 1205387 for more details.
*/
public void testOr4() throws Exception {
testTypes("/**@type {number} */var x;x=null || \"a\";",
"assignment\n" +
"found : string\n" +
"required: number");
}
/**
* @see #testOr4()
*/
public void testOr5() throws Exception {
testTypes("/**@type {number} */var x;x=undefined || \"a\";",
"assignment\n" +
"found : string\n" +
"required: number");
}
public void testAnd1() throws Exception {
testTypes("/** @type number */var a;" +
"/** @type number */var b;" +
"a + b && undefined;");
}
public void testAnd2() throws Exception {
testTypes("/** @type number */var a;" +
"/** @type number */var b;" +
"/** @type number */var c = a + b && undefined;",
"initializing variable\n" +
"found : (number|undefined)\n" +
"required: number");
}
public void testAnd3() throws Exception {
testTypes("/** @type {(!Array, undefined)} */var a;" +
"/** @type number */var c = a && undefined;",
"initializing variable\n" +
"found : undefined\n" +
"required: number");
}
public void testAnd4() throws Exception {
testTypes("/** @param {number} x */function f(x){};\n" +
"/** @type null */var x; /** @type {number?} */var y;\n" +
"if (x && y) { f(y) }");
}
public void testAnd5() throws Exception {
testTypes("/** @param {number} x\n@param {string} y*/function f(x,y){};\n" +
"/** @type {number?} */var x; /** @type {string?} */var y;\n" +
"if (x && y) { f(x, y) }");
}
public void testAnd6() throws Exception {
testTypes("/** @param {number} x */function f(x){};\n" +
"/** @type {number|undefined} */var x;\n" +
"if (x && f(x)) { f(x) }");
}
public void testAnd7() throws Exception {
// TODO(user): a deterministic warning should be generated for this
// case since x && x is always false. The implementation of this requires
// a more precise handling of a null value within a variable's type.
// Currently, a null value defaults to ? which passes every check.
testTypes("/** @type null */var x; if (x && x) {}");
}
public void testHook() throws Exception {
testTypes("/**@return {void}*/function foo(){ var x=foo()?a:b; }");
}
public void testHookRestrictsType1() throws Exception {
testTypes("/** @return {(string,null)} */" +
"function f() { return null;}" +
"/** @type {(string,null)} */ var a = f();" +
"/** @type string */" +
"var b = a ? a : 'default';");
}
public void testHookRestrictsType2() throws Exception {
testTypes("/** @type {String} */" +
"var a = null;" +
"/** @type null */" +
"var b = a ? null : a;");
}
public void testHookRestrictsType3() throws Exception {
testTypes("/** @type {String} */" +
"var a;" +
"/** @type null */" +
"var b = (!a) ? a : null;");
}
public void testHookRestrictsType4() throws Exception {
testTypes("/** @type {(boolean,undefined)} */" +
"var a;" +
"/** @type boolean */" +
"var b = a != null ? a : true;");
}
public void testHookRestrictsType5() throws Exception {
testTypes("/** @type {(boolean,undefined)} */" +
"var a;" +
"/** @type {(undefined)} */" +
"var b = a == null ? a : undefined;");
}
public void testHookRestrictsType6() throws Exception {
testTypes("/** @type {(number,null,undefined)} */" +
"var a;" +
"/** @type {number} */" +
"var b = a == null ? 5 : a;");
}
public void testHookRestrictsType7() throws Exception {
testTypes("/** @type {(number,null,undefined)} */" +
"var a;" +
"/** @type {number} */" +
"var b = a == undefined ? 5 : a;");
}
public void testWhileRestrictsType1() throws Exception {
testTypes("/** @param {null} x */ function g(x) {}" +
"/** @param {number?} x */\n" +
"function f(x) {\n" +
"while (x) {\n" +
"if (g(x)) { x = 1; }\n" +
"x = x-1;\n}\n}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : number\n" +
"required: null");
}
public void testWhileRestrictsType2() throws Exception {
testTypes("/** @param {number?} x\n@return {number}*/\n" +
"function f(x) {\n/** @type {number} */var y = 0;" +
"while (x) {\n" +
"y = x;\n" +
"x = x-1;\n}\n" +
"return y;}");
}
public void testHigherOrderFunctions1() throws Exception {
testTypes(
"/** @type {function(number)} */var f;" +
"f(true);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testHigherOrderFunctions2() throws Exception {
testTypes(
"/** @type {function():!Date} */var f;" +
"/** @type boolean */var a = f();",
"initializing variable\n" +
"found : Date\n" +
"required: boolean");
}
public void testHigherOrderFunctions3() throws Exception {
testTypes(
"/** @type {function(this:Error):Date} */var f; new f",
"cannot instantiate non-constructor");
}
public void testHigherOrderFunctions4() throws Exception {
testTypes(
"/** @type {function(this:Error,...[number]):Date} */var f; new f",
"cannot instantiate non-constructor");
}
public void testHigherOrderFunctions5() throws Exception {
testTypes(
"/** @param {number} x */ function g(x) {}" +
"/** @type {function(new:Error,...[number]):Date} */ var f;" +
"g(new f());",
"actual parameter 1 of g does not match formal parameter\n" +
"found : Error\n" +
"required: number");
}
public void testConstructorAlias1() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {};" +
"/** @type {number} */ Foo.prototype.bar = 3;" +
"/** @constructor */ var FooAlias = Foo;" +
"/** @return {string} */ function foo() { " +
" return (new FooAlias()).bar; }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testConstructorAlias2() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {};" +
"/** @constructor */ var FooAlias = Foo;" +
"/** @type {number} */ FooAlias.prototype.bar = 3;" +
"/** @return {string} */ function foo() { " +
" return (new Foo()).bar; }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testConstructorAlias3() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {};" +
"/** @type {number} */ Foo.prototype.bar = 3;" +
"/** @constructor */ var FooAlias = Foo;" +
"/** @return {string} */ function foo() { " +
" return (new FooAlias()).bar; }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testConstructorAlias4() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {};" +
"var FooAlias = Foo;" +
"/** @type {number} */ FooAlias.prototype.bar = 3;" +
"/** @return {string} */ function foo() { " +
" return (new Foo()).bar; }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testConstructorAlias5() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {};" +
"/** @constructor */ var FooAlias = Foo;" +
"/** @return {FooAlias} */ function foo() { " +
" return new Foo(); }");
}
public void testConstructorAlias6() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {};" +
"/** @constructor */ var FooAlias = Foo;" +
"/** @return {Foo} */ function foo() { " +
" return new FooAlias(); }");
}
public void testConstructorAlias7() throws Exception {
testTypes(
"var goog = {};" +
"/** @constructor */ goog.Foo = function() {};" +
"/** @constructor */ goog.FooAlias = goog.Foo;" +
"/** @return {number} */ function foo() { " +
" return new goog.FooAlias(); }",
"inconsistent return type\n" +
"found : goog.Foo\n" +
"required: number");
}
public void testConstructorAlias8() throws Exception {
testTypes(
"var goog = {};" +
"/**\n * @param {number} x \n * @constructor */ " +
"goog.Foo = function(x) {};" +
"/**\n * @param {number} x \n * @constructor */ " +
"goog.FooAlias = goog.Foo;" +
"/** @return {number} */ function foo() { " +
" return new goog.FooAlias(1); }",
"inconsistent return type\n" +
"found : goog.Foo\n" +
"required: number");
}
public void testConstructorAlias9() throws Exception {
testTypes(
"var goog = {};" +
"/**\n * @param {number} x \n * @constructor */ " +
"goog.Foo = function(x) {};" +
"/** @constructor */ goog.FooAlias = goog.Foo;" +
"/** @return {number} */ function foo() { " +
" return new goog.FooAlias(1); }",
"inconsistent return type\n" +
"found : goog.Foo\n" +
"required: number");
}
public void testConstructorAlias10() throws Exception {
testTypes(
"/**\n * @param {number} x \n * @constructor */ " +
"var Foo = function(x) {};" +
"/** @constructor */ var FooAlias = Foo;" +
"/** @return {number} */ function foo() { " +
" return new FooAlias(1); }",
"inconsistent return type\n" +
"found : Foo\n" +
"required: number");
}
public void testClosure1() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {string|undefined} */var a;" +
"/** @type string */" +
"var b = goog.isDef(a) ? a : 'default';",
null);
}
public void testClosure2() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {string?} */var a;" +
"/** @type string */" +
"var b = goog.isNull(a) ? 'default' : a;",
null);
}
public void testClosure3() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {string|null|undefined} */var a;" +
"/** @type string */" +
"var b = goog.isDefAndNotNull(a) ? a : 'default';",
null);
}
public void testClosure4() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {string|undefined} */var a;" +
"/** @type string */" +
"var b = !goog.isDef(a) ? 'default' : a;",
null);
}
public void testClosure5() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {string?} */var a;" +
"/** @type string */" +
"var b = !goog.isNull(a) ? a : 'default';",
null);
}
public void testClosure6() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {string|null|undefined} */var a;" +
"/** @type string */" +
"var b = !goog.isDefAndNotNull(a) ? 'default' : a;",
null);
}
public void testClosure7() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {string|null|undefined} */ var a = foo();" +
"/** @type {number} */" +
"var b = goog.asserts.assert(a);",
"initializing variable\n" +
"found : string\n" +
"required: number");
}
public void testReturn1() throws Exception {
testTypes("/**@return {void}*/function foo(){ return 3; }",
"inconsistent return type\n" +
"found : number\n" +
"required: undefined");
}
public void testReturn2() throws Exception {
testTypes("/**@return {!Number}*/function foo(){ return; }",
"inconsistent return type\n" +
"found : undefined\n" +
"required: Number");
}
public void testReturn3() throws Exception {
testTypes("/**@return {!Number}*/function foo(){ return 'abc'; }",
"inconsistent return type\n" +
"found : string\n" +
"required: Number");
}
public void testReturn4() throws Exception {
testTypes("/**@return {!Number}\n*/\n function a(){return new Array();}",
"inconsistent return type\n" +
"found : Array\n" +
"required: Number");
}
public void testReturn5() throws Exception {
testTypes("/** @param {number} n\n" +
"@constructor */function n(n){return};");
}
public void testReturn6() throws Exception {
testTypes(
"/** @param {number} opt_a\n@return {string} */" +
"function a(opt_a) { return opt_a }",
"inconsistent return type\n" +
"found : (number|undefined)\n" +
"required: string");
}
public void testReturn7() throws Exception {
testTypes("/** @constructor */var A = function() {};\n" +
"/** @constructor */var B = function() {};\n" +
"/** @return {!B} */A.f = function() { return 1; };",
"inconsistent return type\n" +
"found : number\n" +
"required: B");
}
public void testReturn8() throws Exception {
testTypes("/** @constructor */var A = function() {};\n" +
"/** @constructor */var B = function() {};\n" +
"/** @return {!B} */A.prototype.f = function() { return 1; };",
"inconsistent return type\n" +
"found : number\n" +
"required: B");
}
public void testInferredReturn1() throws Exception {
testTypes(
"function f() {} /** @param {number} x */ function g(x) {}" +
"g(f());",
"actual parameter 1 of g does not match formal parameter\n" +
"found : undefined\n" +
"required: number");
}
public void testInferredReturn2() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() {}; " +
"/** @param {number} x */ function g(x) {}" +
"g((new Foo()).bar());",
"actual parameter 1 of g does not match formal parameter\n" +
"found : undefined\n" +
"required: number");
}
public void testInferredReturn3() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() {}; " +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @return {number} \n * @override */ " +
"SubFoo.prototype.bar = function() { return 3; }; ",
"mismatch of the bar property type and the type of the property " +
"it overrides from superclass Foo\n" +
"original: function (this:Foo): undefined\n" +
"override: function (this:SubFoo): number");
}
public void testInferredReturn4() throws Exception {
// By design, this throws a warning. if you want global x to be
// defined to some other type of function, then you need to declare it
// as a greater type.
testTypes(
"var x = function() {};" +
"x = /** @type {function(): number} */ (function() { return 3; });",
"assignment\n" +
"found : function (): number\n" +
"required: function (): undefined");
}
public void testInferredReturn5() throws Exception {
// If x is local, then the function type is not declared.
testTypes(
"/** @return {string} */" +
"function f() {" +
" var x = function() {};" +
" x = /** @type {function(): number} */ (function() { return 3; });" +
" return x();" +
"}",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testInferredReturn6() throws Exception {
testTypes(
"/** @return {string} */" +
"function f() {" +
" var x = function() {};" +
" if (f()) " +
" x = /** @type {function(): number} */ " +
" (function() { return 3; });" +
" return x();" +
"}",
"inconsistent return type\n" +
"found : (number|undefined)\n" +
"required: string");
}
public void testInferredReturn7() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @param {number} x */ Foo.prototype.bar = function(x) {};" +
"Foo.prototype.bar = function(x) { return 3; };",
"inconsistent return type\n" +
"found : number\n" +
"required: undefined");
}
public void testInferredReturn8() throws Exception {
reportMissingOverrides = CheckLevel.OFF;
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @param {number} x */ Foo.prototype.bar = function(x) {};" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @param {number} x */ SubFoo.prototype.bar = " +
" function(x) { return 3; }",
"inconsistent return type\n" +
"found : number\n" +
"required: undefined");
}
public void testInferredParam1() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @param {number} x */ Foo.prototype.bar = function(x) {};" +
"/** @param {string} x */ function f(x) {}" +
"Foo.prototype.bar = function(y) { f(y); };",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testInferredParam2() throws Exception {
reportMissingOverrides = CheckLevel.OFF;
testTypes(
"/** @param {string} x */ function f(x) {}" +
"/** @constructor */ function Foo() {}" +
"/** @param {number} x */ Foo.prototype.bar = function(x) {};" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @return {void} */ SubFoo.prototype.bar = " +
" function(x) { f(x); }",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testInferredParam3() throws Exception {
reportMissingOverrides = CheckLevel.OFF;
testTypes(
"/** @param {string} x */ function f(x) {}" +
"/** @constructor */ function Foo() {}" +
"/** @param {number=} x */ Foo.prototype.bar = function(x) {};" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @return {void} */ SubFoo.prototype.bar = " +
" function(x) { f(x); }; (new SubFoo()).bar();",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (number|undefined)\n" +
"required: string");
}
public void testInferredParam4() throws Exception {
reportMissingOverrides = CheckLevel.OFF;
testTypes(
"/** @param {string} x */ function f(x) {}" +
"/** @constructor */ function Foo() {}" +
"/** @param {...number} x */ Foo.prototype.bar = function(x) {};" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @return {void} */ SubFoo.prototype.bar = " +
" function(x) { f(x); }; (new SubFoo()).bar();",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (number|undefined)\n" +
"required: string");
}
public void testInferredParam5() throws Exception {
reportMissingOverrides = CheckLevel.OFF;
testTypes(
"/** @param {string} x */ function f(x) {}" +
"/** @constructor */ function Foo() {}" +
"/** @param {...number} x */ Foo.prototype.bar = function(x) {};" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @param {number=} x \n * @param {...number} y */ " +
"SubFoo.prototype.bar = " +
" function(x, y) { f(x); }; (new SubFoo()).bar();",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (number|undefined)\n" +
"required: string");
}
public void testInferredParam6() throws Exception {
reportMissingOverrides = CheckLevel.OFF;
testTypes(
"/** @param {string} x */ function f(x) {}" +
"/** @constructor */ function Foo() {}" +
"/** @param {number=} x */ Foo.prototype.bar = function(x) {};" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @param {number=} x \n * @param {number=} y */ " +
"SubFoo.prototype.bar = " +
" function(x, y) { f(y); };",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (number|undefined)\n" +
"required: string");
}
public void testInferredParam7() throws Exception {
testTypes(
"/** @param {string} x */ function f(x) {}" +
"var bar = /** @type {function(number=,number=)} */ (" +
" function(x, y) { f(y); });",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (number|undefined)\n" +
"required: string");
}
public void testOverriddenParams1() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @param {...?} var_args */" +
"Foo.prototype.bar = function(var_args) {};" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/**\n" +
" * @param {number} x\n" +
" * @override\n" +
" */" +
"SubFoo.prototype.bar = function(x) {};");
}
public void testOverriddenParams2() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @type {function(...[?])} */" +
"Foo.prototype.bar = function(var_args) {};" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/**\n" +
" * @type {function(number)}\n" +
" * @override\n" +
" */" +
"SubFoo.prototype.bar = function(x) {};");
}
public void testOverriddenParams3() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @param {...number} var_args */" +
"Foo.prototype.bar = function(var_args) { };" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/**\n" +
" * @param {number} x\n" +
" * @override\n" +
" */" +
"SubFoo.prototype.bar = function(x) {};",
"mismatch of the bar property type and the type of the " +
"property it overrides from superclass Foo\n" +
"original: function (this:Foo, ...[number]): undefined\n" +
"override: function (this:SubFoo, number): undefined");
}
public void testOverriddenParams4() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @type {function(...[number])} */" +
"Foo.prototype.bar = function(var_args) {};" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/**\n" +
" * @type {function(number)}\n" +
" * @override\n" +
" */" +
"SubFoo.prototype.bar = function(x) {};",
"mismatch of the bar property type and the type of the " +
"property it overrides from superclass Foo\n" +
"original: function (...[number]): ?\n" +
"override: function (number): ?");
}
public void testOverriddenParams5() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @param {number} x */" +
"Foo.prototype.bar = function(x) { };" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/**\n" +
" * @override\n" +
" */" +
"SubFoo.prototype.bar = function() {};" +
"(new SubFoo()).bar();");
}
public void testOverriddenParams6() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @param {number} x */" +
"Foo.prototype.bar = function(x) { };" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/**\n" +
" * @override\n" +
" */" +
"SubFoo.prototype.bar = function() {};" +
"(new SubFoo()).bar(true);",
"actual parameter 1 of SubFoo.prototype.bar " +
"does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testOverriddenReturn1() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @return {Object} */ Foo.prototype.bar = " +
" function() { return {}; };" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @return {SubFoo}\n * @override */ SubFoo.prototype.bar = " +
" function() { return new Foo(); }",
"inconsistent return type\n" +
"found : Foo\n" +
"required: (SubFoo|null)");
}
public void testOverriddenReturn2() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @return {SubFoo} */ Foo.prototype.bar = " +
" function() { return new SubFoo(); };" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"/** @return {Foo} x\n * @override */ SubFoo.prototype.bar = " +
" function() { return new SubFoo(); }",
"mismatch of the bar property type and the type of the " +
"property it overrides from superclass Foo\n" +
"original: function (this:Foo): (SubFoo|null)\n" +
"override: function (this:SubFoo): (Foo|null)");
}
public void testThis1() throws Exception {
testTypes("var goog = {};" +
"/** @constructor */goog.A = function(){};" +
"/** @return {number} */" +
"goog.A.prototype.n = function() { return this };",
"inconsistent return type\n" +
"found : goog.A\n" +
"required: number");
}
public void testOverriddenProperty1() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @type {Object} */" +
"Foo.prototype.bar = {};" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/**\n" +
" * @type {Array}\n" +
" * @override\n" +
" */" +
"SubFoo.prototype.bar = [];");
}
public void testOverriddenProperty2() throws Exception {
testTypes(
"/** @constructor */ function Foo() {" +
" /** @type {Object} */" +
" this.bar = {};" +
"}" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/**\n" +
" * @type {Array}\n" +
" * @override\n" +
" */" +
"SubFoo.prototype.bar = [];");
}
public void testOverriddenProperty3() throws Exception {
testTypes(
"/** @constructor */ function Foo() {" +
"}" +
"/** @type {string} */ Foo.prototype.data;" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/** @type {string|Object} \n @override */ " +
"SubFoo.prototype.data = null;",
"mismatch of the data property type and the type " +
"of the property it overrides from superclass Foo\n" +
"original: string\n" +
"override: (Object|null|string)");
}
public void testOverriddenProperty4() throws Exception {
// These properties aren't declared, so there should be no warning.
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = null;" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"SubFoo.prototype.bar = 3;");
}
public void testOverriddenProperty5() throws Exception {
// An override should be OK if the superclass property wasn't declared.
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = null;" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/** @override */ SubFoo.prototype.bar = 3;");
}
public void testOverriddenProperty6() throws Exception {
// The override keyword shouldn't be neccessary if the subclass property
// is inferred.
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @type {?number} */ Foo.prototype.bar = null;" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"SubFoo.prototype.bar = 3;");
}
public void testThis2() throws Exception {
testTypes("var goog = {};" +
"/** @constructor */goog.A = function(){" +
" this.foo = null;" +
"};" +
"/** @return {number} */" +
"goog.A.prototype.n = function() { return this.foo };",
"inconsistent return type\n" +
"found : null\n" +
"required: number");
}
public void testThis3() throws Exception {
testTypes("var goog = {};" +
"/** @constructor */goog.A = function(){" +
" this.foo = null;" +
" this.foo = 5;" +
"};");
}
public void testThis4() throws Exception {
testTypes("var goog = {};" +
"/** @constructor */goog.A = function(){" +
" /** @type {string?} */this.foo = null;" +
"};" +
"/** @return {number} */goog.A.prototype.n = function() {" +
" return this.foo };",
"inconsistent return type\n" +
"found : (null|string)\n" +
"required: number");
}
public void testThis5() throws Exception {
testTypes("/** @this Date\n@return {number}*/function h() { return this }",
"inconsistent return type\n" +
"found : Date\n" +
"required: number");
}
public void testThis6() throws Exception {
testTypes("var goog = {};" +
"/** @constructor\n@return {!Date} */" +
"goog.A = function(){ return this };",
"inconsistent return type\n" +
"found : goog.A\n" +
"required: Date");
}
public void testThis7() throws Exception {
testTypes("/** @constructor */function A(){};" +
"/** @return {number} */A.prototype.n = function() { return this };",
"inconsistent return type\n" +
"found : A\n" +
"required: number");
}
public void testThis8() throws Exception {
testTypes("/** @constructor */function A(){" +
" /** @type {string?} */this.foo = null;" +
"};" +
"/** @return {number} */A.prototype.n = function() {" +
" return this.foo };",
"inconsistent return type\n" +
"found : (null|string)\n" +
"required: number");
}
public void testThis9() throws Exception {
// In A.bar, the type of {@code this} is unknown.
testTypes("/** @constructor */function A(){};" +
"A.prototype.foo = 3;" +
"/** @return {string} */ A.bar = function() { return this.foo; };");
}
public void testThis10() throws Exception {
// In A.bar, the type of {@code this} is inferred from the @this tag.
testTypes("/** @constructor */function A(){};" +
"A.prototype.foo = 3;" +
"/** @this {A}\n@return {string} */" +
"A.bar = function() { return this.foo; };",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testThis11() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"/** @constructor */ function Ctor() {" +
" /** @this {Date} */" +
" this.method = function() {" +
" f(this);" +
" };" +
"}",
"actual parameter 1 of f does not match formal parameter\n" +
"found : Date\n" +
"required: number");
}
public void testThis12() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"/** @constructor */ function Ctor() {}" +
"Ctor.prototype['method'] = function() {" +
" f(this);" +
"}",
"actual parameter 1 of f does not match formal parameter\n" +
"found : Ctor\n" +
"required: number");
}
public void testThis13() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"/** @constructor */ function Ctor() {}" +
"Ctor.prototype = {" +
" method: function() {" +
" f(this);" +
" }" +
"};",
"actual parameter 1 of f does not match formal parameter\n" +
"found : Ctor\n" +
"required: number");
}
public void testThis14() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"f(this.Object);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : function (new:Object, *=): ?\n" +
"required: number");
}
public void testThisTypeOfFunction1() throws Exception {
testTypes(
"/** @type {function(this:Object)} */ function f() {}" +
"f();");
}
public void testThisTypeOfFunction2() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** @type {function(this:F)} */ function f() {}" +
"f();",
"\"function (this:F): ?\" must be called with a \"this\" type");
}
public void testThisTypeOfFunction3() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"F.prototype.bar = function() {};" +
"var f = (new F()).bar; f();",
"\"function (this:F): undefined\" must be called with a \"this\" type");
}
public void testThisTypeOfFunction4() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"F.prototype.moveTo = function(x, y) {};" +
"F.prototype.lineTo = function(x, y) {};" +
"function demo() {" +
" var path = new F();" +
" var points = [[1,1], [2,2]];" +
" for (var i = 0; i < points.length; i++) {" +
" (i == 0 ? path.moveTo : path.lineTo)(" +
" points[i][0], points[i][1]);" +
" }" +
"}",
"\"function (this:F, ?, ?): undefined\" " +
"must be called with a \"this\" type");
}
public void testGlobalThis1() throws Exception {
testTypes("/** @constructor */ function Window() {}" +
"/** @param {string} msg */ " +
"Window.prototype.alert = function(msg) {};" +
"this.alert(3);",
"actual parameter 1 of Window.prototype.alert " +
"does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testGlobalThis2() throws Exception {
// this.alert = 3 doesn't count as a declaration, so this isn't a warning.
testTypes("/** @constructor */ function Bindow() {}" +
"/** @param {string} msg */ " +
"Bindow.prototype.alert = function(msg) {};" +
"this.alert = 3;" +
"(new Bindow()).alert(this.alert)");
}
public void testGlobalThis2b() throws Exception {
testTypes("/** @constructor */ function Bindow() {}" +
"/** @param {string} msg */ " +
"Bindow.prototype.alert = function(msg) {};" +
"/** @return {number} */ this.alert = function() { return 3; };" +
"(new Bindow()).alert(this.alert())",
"actual parameter 1 of Bindow.prototype.alert " +
"does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testGlobalThis3() throws Exception {
testTypes(
"/** @param {string} msg */ " +
"function alert(msg) {};" +
"this.alert(3);",
"actual parameter 1 of global this.alert " +
"does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testGlobalThis4() throws Exception {
testTypes(
"/** @param {string} msg */ " +
"var alert = function(msg) {};" +
"this.alert(3);",
"actual parameter 1 of global this.alert " +
"does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testGlobalThis5() throws Exception {
testTypes(
"function f() {" +
" /** @param {string} msg */ " +
" var alert = function(msg) {};" +
"}" +
"this.alert(3);",
"Property alert never defined on global this");
}
public void testGlobalThis6() throws Exception {
testTypes(
"/** @param {string} msg */ " +
"var alert = function(msg) {};" +
"var x = 3;" +
"x = 'msg';" +
"this.alert(this.x);");
}
public void testGlobalThis7() throws Exception {
testTypes(
"/** @constructor */ function Window() {}" +
"/** @param {Window} msg */ " +
"var foo = function(msg) {};" +
"foo(this);");
}
public void testGlobalThis8() throws Exception {
testTypes(
"/** @constructor */ function Window() {}" +
"/** @param {number} msg */ " +
"var foo = function(msg) {};" +
"foo(this);",
"actual parameter 1 of foo does not match formal parameter\n" +
"found : global this\n" +
"required: number");
}
public void testGlobalThis9() throws Exception {
testTypes(
// Window is not marked as a constructor, so the
// inheritance doesn't happen.
"function Window() {}" +
"Window.prototype.alert = function() {};" +
"this.alert();",
"Property alert never defined on global this");
}
public void testControlFlowRestrictsType1() throws Exception {
testTypes("/** @return {String?} */ function f() { return null; }" +
"/** @type {String?} */ var a = f();" +
"/** @type String */ var b = new String('foo');" +
"/** @type null */ var c = null;" +
"if (a) {" +
" b = a;" +
"} else {" +
" c = a;" +
"}");
}
public void testControlFlowRestrictsType2() throws Exception {
testTypes("/** @return {(string,null)} */ function f() { return null; }" +
"/** @type {(string,null)} */ var a = f();" +
"/** @type string */ var b = 'foo';" +
"/** @type null */ var c = null;" +
"if (a) {" +
" b = a;" +
"} else {" +
" c = a;" +
"}",
"assignment\n" +
"found : (null|string)\n" +
"required: null");
}
public void testControlFlowRestrictsType3() throws Exception {
testTypes("/** @type {(string,void)} */" +
"var a;" +
"/** @type string */" +
"var b = 'foo';" +
"if (a) {" +
" b = a;" +
"}");
}
public void testControlFlowRestrictsType4() throws Exception {
testTypes("/** @param {string} a */ function f(a){}" +
"/** @type {(string,undefined)} */ var a;" +
"a && f(a);");
}
public void testControlFlowRestrictsType5() throws Exception {
testTypes("/** @param {undefined} a */ function f(a){}" +
"/** @type {(!Array,undefined)} */ var a;" +
"a || f(a);");
}
public void testControlFlowRestrictsType6() throws Exception {
testTypes("/** @param {undefined} x */ function f(x) {}" +
"/** @type {(string,undefined)} */ var a;" +
"a && f(a);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: undefined");
}
public void testControlFlowRestrictsType7() throws Exception {
testTypes("/** @param {undefined} x */ function f(x) {}" +
"/** @type {(string,undefined)} */ var a;" +
"a && f(a);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: undefined");
}
public void testControlFlowRestrictsType8() throws Exception {
testTypes("/** @param {undefined} a */ function f(a){}" +
"/** @type {(!Array,undefined)} */ var a;" +
"if (a || f(a)) {}");
}
public void testControlFlowRestrictsType9() throws Exception {
testTypes("/** @param {number?} x\n * @return {number}*/\n" +
"var f = function(x) {\n" +
"if (!x || x == 1) { return 1; } else { return x; }\n" +
"};");
}
public void testControlFlowRestrictsType10() throws Exception {
// We should correctly infer that y will be (null|{}) because
// the loop wraps around.
testTypes("/** @param {number} x */ function f(x) {}" +
"function g() {" +
" var y = null;" +
" for (var i = 0; i < 10; i++) {" +
" f(y);" +
" if (y != null) {" +
" // y is None the first time it goes through this branch\n" +
" } else {" +
" y = {};" +
" }" +
" }" +
"};",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (null|{})\n" +
"required: number");
}
public void testControlFlowRestrictsType11() throws Exception {
testTypes("/** @param {boolean} x */ function f(x) {}" +
"function g() {" +
" var y = null;" +
" if (y != null) {" +
" for (var i = 0; i < 10; i++) {" +
" f(y);" +
" }" +
" }" +
"};",
"condition always evaluates to false\n" +
"left : null\n" +
"right: null");
}
public void testSwitchCase3() throws Exception {
testTypes("/** @type String */" +
"var a = new String('foo');" +
"switch (a) { case 'A': }");
}
public void testSwitchCase4() throws Exception {
testTypes("/** @type {(string,Null)} */" +
"var a = 'foo';" +
"switch (a) { case 'A':break; case null:break; }");
}
public void testSwitchCase5() throws Exception {
testTypes("/** @type {(String,Null)} */" +
"var a = new String('foo');" +
"switch (a) { case 'A':break; case null:break; }");
}
public void testSwitchCase6() throws Exception {
testTypes("/** @type {(Number,Null)} */" +
"var a = new Number(5);" +
"switch (a) { case 5:break; case null:break; }");
}
public void testSwitchCase7() throws Exception {
// This really tests the inference inside the case.
testTypes(
"/**\n" +
" * @param {number} x\n" +
" * @return {number}\n" +
" */\n" +
"function g(x) { return 5; }" +
"function f() {" +
" var x = {};" +
" x.foo = '3';" +
" switch (3) { case g(x.foo): return 3; }" +
"}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testSwitchCase8() throws Exception {
// This really tests the inference inside the switch clause.
testTypes(
"/**\n" +
" * @param {number} x\n" +
" * @return {number}\n" +
" */\n" +
"function g(x) { return 5; }" +
"function f() {" +
" var x = {};" +
" x.foo = '3';" +
" switch (g(x.foo)) { case 3: return 3; }" +
"}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testNoTypeCheck1() throws Exception {
testTypes("/** @notypecheck */function foo() { new 4 }");
}
public void testNoTypeCheck2() throws Exception {
testTypes("/** @notypecheck */var foo = function() { new 4 }");
}
public void testNoTypeCheck3() throws Exception {
testTypes("/** @notypecheck */var foo = function bar() { new 4 }");
}
public void testNoTypeCheck4() throws Exception {
testTypes("var foo;" +
"/** @notypecheck */foo = function() { new 4 }");
}
public void testNoTypeCheck5() throws Exception {
testTypes("var foo;" +
"foo = /** @notypecheck */function() { new 4 }");
}
public void testNoTypeCheck6() throws Exception {
testTypes("var foo;" +
"/** @notypecheck */foo = function bar() { new 4 }");
}
public void testNoTypeCheck7() throws Exception {
testTypes("var foo;" +
"foo = /** @notypecheck */function bar() { new 4 }");
}
public void testNoTypeCheck8() throws Exception {
testTypes("/** @fileoverview \n * @notypecheck */ var foo;" +
"var bar = 3; /** @param {string} x */ function f(x) {} f(bar);");
}
public void testNoTypeCheck9() throws Exception {
testTypes("/** @notypecheck */ function g() { }" +
" /** @type {string} */ var a = 1",
"initializing variable\n" +
"found : number\n" +
"required: string"
);
}
public void testNoTypeCheck10() throws Exception {
testTypes("/** @notypecheck */ function g() { }" +
" function h() {/** @type {string} */ var a = 1}",
"initializing variable\n" +
"found : number\n" +
"required: string"
);
}
public void testNoTypeCheck11() throws Exception {
testTypes("/** @notypecheck */ function g() { }" +
"/** @notypecheck */ function h() {/** @type {string} */ var a = 1}"
);
}
public void testNoTypeCheck12() throws Exception {
testTypes("/** @notypecheck */ function g() { }" +
"function h() {/** @type {string}\n * @notypecheck\n*/ var a = 1}"
);
}
public void testNoTypeCheck13() throws Exception {
testTypes("/** @notypecheck */ function g() { }" +
"function h() {/** @type {string}\n * @notypecheck\n*/ var a = 1;" +
"/** @type {string}*/ var b = 1}",
"initializing variable\n" +
"found : number\n" +
"required: string"
);
}
public void testNoTypeCheck14() throws Exception {
testTypes("/** @fileoverview \n * @notypecheck */ function g() { }" +
"g(1,2,3)");
}
public void testImplicitCast() throws Exception {
testTypes("/** @constructor */ function Element() {};\n" +
"/** @type {string}\n" +
" * @implicitCast */" +
"Element.prototype.innerHTML;",
"(new Element).innerHTML = new Array();", null, false);
}
public void testImplicitCastSubclassAccess() throws Exception {
testTypes("/** @constructor */ function Element() {};\n" +
"/** @type {string}\n" +
" * @implicitCast */" +
"Element.prototype.innerHTML;" +
"/** @constructor \n @extends Element */" +
"function DIVElement() {};",
"(new DIVElement).innerHTML = new Array();",
null, false);
}
public void testImplicitCastNotInExterns() throws Exception {
testTypes("/** @constructor */ function Element() {};\n" +
"/** @type {string}\n" +
" * @implicitCast */" +
"Element.prototype.innerHTML;" +
"(new Element).innerHTML = new Array();",
new String[] {
"Illegal annotation on innerHTML. @implicitCast may only be " +
"used in externs.",
"assignment to property innerHTML of Element\n" +
"found : Array\n" +
"required: string"});
}
public void testNumberNode() throws Exception {
Node n = typeCheck(Node.newNumber(0));
assertTypeEquals(NUMBER_TYPE, n.getJSType());
}
public void testStringNode() throws Exception {
Node n = typeCheck(Node.newString("hello"));
assertTypeEquals(STRING_TYPE, n.getJSType());
}
public void testBooleanNodeTrue() throws Exception {
Node trueNode = typeCheck(new Node(Token.TRUE));
assertTypeEquals(BOOLEAN_TYPE, trueNode.getJSType());
}
public void testBooleanNodeFalse() throws Exception {
Node falseNode = typeCheck(new Node(Token.FALSE));
assertTypeEquals(BOOLEAN_TYPE, falseNode.getJSType());
}
public void testUndefinedNode() throws Exception {
Node p = new Node(Token.ADD);
Node n = Node.newString(Token.NAME, "undefined");
p.addChildToBack(n);
p.addChildToBack(Node.newNumber(5));
typeCheck(p);
assertTypeEquals(VOID_TYPE, n.getJSType());
}
public void testNumberAutoboxing() throws Exception {
testTypes("/** @type Number */var a = 4;",
"initializing variable\n" +
"found : number\n" +
"required: (Number|null)");
}
public void testNumberUnboxing() throws Exception {
testTypes("/** @type number */var a = new Number(4);",
"initializing variable\n" +
"found : Number\n" +
"required: number");
}
public void testStringAutoboxing() throws Exception {
testTypes("/** @type String */var a = 'hello';",
"initializing variable\n" +
"found : string\n" +
"required: (String|null)");
}
public void testStringUnboxing() throws Exception {
testTypes("/** @type string */var a = new String('hello');",
"initializing variable\n" +
"found : String\n" +
"required: string");
}
public void testBooleanAutoboxing() throws Exception {
testTypes("/** @type Boolean */var a = true;",
"initializing variable\n" +
"found : boolean\n" +
"required: (Boolean|null)");
}
public void testBooleanUnboxing() throws Exception {
testTypes("/** @type boolean */var a = new Boolean(false);",
"initializing variable\n" +
"found : Boolean\n" +
"required: boolean");
}
public void testIIFE1() throws Exception {
testTypes(
"var namespace = {};" +
"/** @type {number} */ namespace.prop = 3;" +
"(function(ns) {" +
" ns.prop = true;" +
"})(namespace);",
"assignment to property prop of ns\n" +
"found : boolean\n" +
"required: number");
}
public void testIIFE2() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"(function(ctor) {" +
" /** @type {boolean} */ ctor.prop = true;" +
"})(Foo);" +
"/** @return {number} */ function f() { return Foo.prop; }",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testIIFE3() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"(function(ctor) {" +
" /** @type {boolean} */ ctor.prop = true;" +
"})(Foo);" +
"/** @param {number} x */ function f(x) {}" +
"f(Foo.prop);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testIIFE4() throws Exception {
testTypes(
"/** @const */ var namespace = {};" +
"(function(ns) {" +
" /**\n" +
" * @constructor\n" +
" * @param {number} x\n" +
" */\n" +
" ns.Ctor = function(x) {};" +
"})(namespace);" +
"new namespace.Ctor(true);",
"actual parameter 1 of namespace.Ctor " +
"does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testIIFE5() throws Exception {
// TODO(nicksantos): This behavior is currently incorrect.
// To handle this case properly, we'll need to change how we handle
// type resolution.
testTypes(
"/** @const */ var namespace = {};" +
"(function(ns) {" +
" /**\n" +
" * @constructor\n" +
" */\n" +
" ns.Ctor = function() {};" +
" /** @type {boolean} */ ns.Ctor.prototype.bar = true;" +
"})(namespace);" +
"/** @param {namespace.Ctor} x\n" +
" * @return {number} */ function f(x) { return x.bar; }",
"Bad type annotation. Unknown type namespace.Ctor");
}
public void testNotIIFE1() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"/** @param {...?} x */ function g(x) {}" +
"g(function(y) { f(y); }, true);");
}
public void testIssue61() throws Exception {
testTypes(
"var ns = {};" +
"(function() {" +
" /** @param {string} b */" +
" ns.a = function(b) {};" +
"})();" +
"function d() {" +
" ns.a(123);" +
"}",
"actual parameter 1 of ns.a does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testIssue61b() throws Exception {
testTypes(
"var ns = {};" +
"(function() {" +
" /** @param {string} b */" +
" ns.a = function(b) {};" +
"})();" +
"ns.a(123);",
"actual parameter 1 of ns.a does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testIssue86() throws Exception {
testTypes(
"/** @interface */ function I() {}" +
"/** @return {number} */ I.prototype.get = function(){};" +
"/** @constructor \n * @implements {I} */ function F() {}" +
"/** @override */ F.prototype.get = function() { return true; };",
"inconsistent return type\n" +
"found : boolean\n" +
"required: number");
}
public void testIssue124() throws Exception {
testTypes(
"var t = null;" +
"function test() {" +
" if (t != null) { t = null; }" +
" t = 1;" +
"}");
}
public void testIssue124b() throws Exception {
testTypes(
"var t = null;" +
"function test() {" +
" if (t != null) { t = null; }" +
" t = undefined;" +
"}",
"condition always evaluates to false\n" +
"left : (null|undefined)\n" +
"right: null");
}
public void testIssue259() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"/** @constructor */" +
"var Clock = function() {" +
" /** @constructor */" +
" this.Date = function() {};" +
" f(new this.Date());" +
"};",
"actual parameter 1 of f does not match formal parameter\n" +
"found : this.Date\n" +
"required: number");
}
public void testIssue301() throws Exception {
testTypes(
"Array.indexOf = function() {};" +
"var s = 'hello';" +
"alert(s.toLowerCase.indexOf('1'));",
"Property indexOf never defined on String.prototype.toLowerCase");
}
public void testIssue368() throws Exception {
testTypes(
"/** @constructor */ function Foo(){}" +
"/**\n" +
" * @param {number} one\n" +
" * @param {string} two\n" +
" */\n" +
"Foo.prototype.add = function(one, two) {};" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */\n" +
"function Bar(){}" +
"/** @override */\n" +
"Bar.prototype.add = function(ignored) {};" +
"(new Bar()).add(1, 2);",
"actual parameter 2 of Bar.prototype.add does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testIssue380() throws Exception {
testTypes(
"/** @type { function(string): {innerHTML: string} } */\n" +
"document.getElementById;\n" +
"var list = /** @type {!Array.<string>} */ ['hello', 'you'];\n" +
"list.push('?');\n" +
"document.getElementById('node').innerHTML = list.toString();",
// Parse warning, but still applied.
"Type annotations are not allowed here. " +
"Are you missing parentheses?");
}
public void testIssue483() throws Exception {
testTypes(
"/** @constructor */ function C() {" +
" /** @type {?Array} */ this.a = [];" +
"}" +
"C.prototype.f = function() {" +
" if (this.a.length > 0) {" +
" g(this.a);" +
" }" +
"};" +
"/** @param {number} a */ function g(a) {}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : Array\n" +
"required: number");
}
public void testIssue537a() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype = {method: function() {}};" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */\n" +
"function Bar() {" +
" Foo.call(this);" +
" if (this.baz()) this.method(1);" +
"}" +
"Bar.prototype = {" +
" baz: function() {" +
" return true;" +
" }" +
"};" +
"Bar.prototype.__proto__ = Foo.prototype;",
"Function Foo.prototype.method: called with 1 argument(s). " +
"Function requires at least 0 argument(s) " +
"and no more than 0 argument(s).");
}
public void testIssue537b() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype = {method: function() {}};" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */\n" +
"function Bar() {" +
" Foo.call(this);" +
" if (this.baz(1)) this.method();" +
"}" +
"Bar.prototype = {" +
" baz: function() {" +
" return true;" +
" }" +
"};" +
"Bar.prototype.__proto__ = Foo.prototype;",
"Function Bar.prototype.baz: called with 1 argument(s). " +
"Function requires at least 0 argument(s) " +
"and no more than 0 argument(s).");
}
public void testIssue537c() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */\n" +
"function Bar() {" +
" Foo.call(this);" +
" if (this.baz2()) alert(1);" +
"}" +
"Bar.prototype = {" +
" baz: function() {" +
" return true;" +
" }" +
"};" +
"Bar.prototype.__proto__ = Foo.prototype;",
"Property baz2 never defined on Bar");
}
public void testIssue537d() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype = {" +
" /** @return {Bar} */ x: function() { new Bar(); }," +
" /** @return {Foo} */ y: function() { new Bar(); }" +
"};" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */\n" +
"function Bar() {" +
" this.xy = 3;" +
"}" +
"/** @return {Bar} */ function f() { return new Bar(); }" +
"/** @return {Foo} */ function g() { return new Bar(); }" +
"Bar.prototype = {" +
" /** @return {Bar} */ x: function() { new Bar(); }," +
" /** @return {Foo} */ y: function() { new Bar(); }" +
"};" +
"Bar.prototype.__proto__ = Foo.prototype;");
}
public void testIssue586() throws Exception {
testTypes(
"/** @constructor */" +
"var MyClass = function() {};" +
"/** @param {boolean} success */" +
"MyClass.prototype.fn = function(success) {};" +
"MyClass.prototype.test = function() {" +
" this.fn();" +
" this.fn = function() {};" +
"};",
"Function MyClass.prototype.fn: called with 0 argument(s). " +
"Function requires at least 1 argument(s) " +
"and no more than 1 argument(s).");
}
public void testIssue635() throws Exception {
// TODO(nicksantos): Make this emit a warning, because of the 'this' type.
testTypes(
"/** @constructor */" +
"function F() {}" +
"F.prototype.bar = function() { this.baz(); };" +
"F.prototype.baz = function() {};" +
"/** @constructor */" +
"function G() {}" +
"G.prototype.bar = F.prototype.bar;");
}
public void testIssue635b() throws Exception {
testTypes(
"/** @constructor */" +
"function F() {}" +
"/** @constructor */" +
"function G() {}" +
"/** @type {function(new:G)} */ var x = F;",
"initializing variable\n" +
"found : function (new:F): undefined\n" +
"required: function (new:G): ?");
}
public void testIssue669() throws Exception {
testTypes(
"/** @return {{prop1: (Object|undefined)}} */" +
"function f(a) {" +
" var results;" +
" if (a) {" +
" results = {};" +
" results.prop1 = {a: 3};" +
" } else {" +
" results = {prop2: 3};" +
" }" +
" return results;" +
"}");
}
public void testIssue688() throws Exception {
testTypes(
"/** @const */ var SOME_DEFAULT =\n" +
" /** @type {TwoNumbers} */ ({first: 1, second: 2});\n" +
"/**\n" +
"* Class defining an interface with two numbers.\n" +
"* @interface\n" +
"*/\n" +
"function TwoNumbers() {}\n" +
"/** @type number */\n" +
"TwoNumbers.prototype.first;\n" +
"/** @type number */\n" +
"TwoNumbers.prototype.second;\n" +
"/** @return {number} */ function f() { return SOME_DEFAULT; }",
"inconsistent return type\n" +
"found : (TwoNumbers|null)\n" +
"required: number");
}
public void testIssue700() throws Exception {
testTypes(
"/**\n" +
" * @param {{text: string}} opt_data\n" +
" * @return {string}\n" +
" */\n" +
"function temp1(opt_data) {\n" +
" return opt_data.text;\n" +
"}\n" +
"\n" +
"/**\n" +
" * @param {{activity: (boolean|number|string|null|Object)}} opt_data\n" +
" * @return {string}\n" +
" */\n" +
"function temp2(opt_data) {\n" +
" /** @notypecheck */\n" +
" function __inner() {\n" +
" return temp1(opt_data.activity);\n" +
" }\n" +
" return __inner();\n" +
"}\n" +
"\n" +
"/**\n" +
" * @param {{n: number, text: string, b: boolean}} opt_data\n" +
" * @return {string}\n" +
" */\n" +
"function temp3(opt_data) {\n" +
" return 'n: ' + opt_data.n + ', t: ' + opt_data.text + '.';\n" +
"}\n" +
"\n" +
"function callee() {\n" +
" var output = temp3({\n" +
" n: 0,\n" +
" text: 'a string',\n" +
" b: true\n" +
" })\n" +
" alert(output);\n" +
"}\n" +
"\n" +
"callee();");
}
public void testIssue725() throws Exception {
testTypes(
"/** @typedef {{name: string}} */ var RecordType1;" +
"/** @typedef {{name2: string}} */ var RecordType2;" +
"/** @param {RecordType1} rec */ function f(rec) {" +
" alert(rec.name2);" +
"}",
"Property name2 never defined on rec");
}
public void testIssue726() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @param {number} x */ Foo.prototype.bar = function(x) {};" +
"/** @return {!Function} */ " +
"Foo.prototype.getDeferredBar = function() { " +
" var self = this;" +
" return function() {" +
" self.bar(true);" +
" };" +
"};",
"actual parameter 1 of Foo.prototype.bar does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testIssue765() throws Exception {
testTypes(
"/** @constructor */" +
"var AnotherType = function (parent) {" +
" /** @param {string} stringParameter Description... */" +
" this.doSomething = function (stringParameter) {};" +
"};" +
"/** @constructor */" +
"var YetAnotherType = function () {" +
" this.field = new AnotherType(self);" +
" this.testfun=function(stringdata) {" +
" this.field.doSomething(null);" +
" };" +
"};",
"actual parameter 1 of AnotherType.doSomething " +
"does not match formal parameter\n" +
"found : null\n" +
"required: string");
}
public void testIssue783() throws Exception {
testTypes(
"/** @constructor */" +
"var Type = function () {" +
" /** @type {Type} */" +
" this.me_ = this;" +
"};" +
"Type.prototype.doIt = function() {" +
" var me = this.me_;" +
" for (var i = 0; i < me.unknownProp; i++) {}" +
"};",
"Property unknownProp never defined on Type");
}
public void testIssue791() throws Exception {
testTypes(
"/** @param {{func: function()}} obj */" +
"function test1(obj) {}" +
"var fnStruc1 = {};" +
"fnStruc1.func = function() {};" +
"test1(fnStruc1);");
}
public void testIssue810() throws Exception {
testTypes(
"/** @constructor */" +
"var Type = function () {" +
"};" +
"Type.prototype.doIt = function(obj) {" +
" this.prop = obj.unknownProp;" +
"};",
"Property unknownProp never defined on obj");
}
/**
* Tests that the || operator is type checked correctly, that is of
* the type of the first argument or of the second argument. See
* bugid 592170 for more details.
*/
public void testBug592170() throws Exception {
testTypes(
"/** @param {Function} opt_f ... */" +
"function foo(opt_f) {" +
" /** @type {Function} */" +
" return opt_f || function () {};" +
"}",
"Type annotations are not allowed here. Are you missing parentheses?");
}
/**
* Tests that undefined can be compared shallowly to a value of type
* (number,undefined) regardless of the side on which the undefined
* value is.
*/
public void testBug901455() throws Exception {
testTypes("/** @return {(number,undefined)} */ function a() { return 3; }" +
"var b = undefined === a()");
testTypes("/** @return {(number,undefined)} */ function a() { return 3; }" +
"var b = a() === undefined");
}
/**
* Tests that the match method of strings returns nullable arrays.
*/
public void testBug908701() throws Exception {
testTypes("/** @type {String} */var s = new String('foo');" +
"var b = s.match(/a/) != null;");
}
/**
* Tests that named types play nicely with subtyping.
*/
public void testBug908625() throws Exception {
testTypes("/** @constructor */function A(){}" +
"/** @constructor\n * @extends A */function B(){}" +
"/** @param {B} b" +
"\n @return {(A,undefined)} */function foo(b){return b}");
}
/**
* Tests that assigning two untyped functions to a variable whose type is
* inferred and calling this variable is legal.
*/
public void testBug911118() throws Exception {
// verifying the type assigned to function expressions assigned variables
Scope s = parseAndTypeCheckWithScope("var a = function(){};").scope;
JSType type = s.getVar("a").getType();
assertEquals("function (): undefined", type.toString());
// verifying the bug example
testTypes("function nullFunction() {};" +
"var foo = nullFunction;" +
"foo = function() {};" +
"foo();");
}
public void testBug909000() throws Exception {
testTypes("/** @constructor */function A(){}\n" +
"/** @param {!A} a\n" +
"@return {boolean}*/\n" +
"function y(a) { return a }",
"inconsistent return type\n" +
"found : A\n" +
"required: boolean");
}
public void testBug930117() throws Exception {
testTypes(
"/** @param {boolean} x */function f(x){}" +
"f(null);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : null\n" +
"required: boolean");
}
public void testBug1484445() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @type {number?} */ Foo.prototype.bar = null;" +
"/** @type {number?} */ Foo.prototype.baz = null;" +
"/** @param {Foo} foo */" +
"function f(foo) {" +
" while (true) {" +
" if (foo.bar == null && foo.baz == null) {" +
" foo.bar;" +
" }" +
" }" +
"}");
}
public void testBug1859535() throws Exception {
testTypes(
"/**\n" +
" * @param {Function} childCtor Child class.\n" +
" * @param {Function} parentCtor Parent class.\n" +
" */" +
"var inherits = function(childCtor, parentCtor) {" +
" /** @constructor */" +
" function tempCtor() {};" +
" tempCtor.prototype = parentCtor.prototype;" +
" childCtor.superClass_ = parentCtor.prototype;" +
" childCtor.prototype = new tempCtor();" +
" /** @override */ childCtor.prototype.constructor = childCtor;" +
"};" +
"/**" +
" * @param {Function} constructor\n" +
" * @param {Object} var_args\n" +
" * @return {Object}\n" +
" */" +
"var factory = function(constructor, var_args) {" +
" /** @constructor */" +
" var tempCtor = function() {};" +
" tempCtor.prototype = constructor.prototype;" +
" var obj = new tempCtor();" +
" constructor.apply(obj, arguments);" +
" return obj;" +
"};");
}
public void testBug1940591() throws Exception {
testTypes(
"/** @type {Object} */" +
"var a = {};\n" +
"/** @type {number} */\n" +
"a.name = 0;\n" +
"/**\n" +
" * @param {Function} x anything.\n" +
" */\n" +
"a.g = function(x) { x.name = 'a'; }");
}
public void testBug1942972() throws Exception {
testTypes(
"var google = {\n" +
" gears: {\n" +
" factory: {},\n" +
" workerPool: {}\n" +
" }\n" +
"};\n" +
"\n" +
"google.gears = {factory: {}};\n");
}
public void testBug1943776() throws Exception {
testTypes(
"/** @return {{foo: Array}} */" +
"function bar() {" +
" return {foo: []};" +
"}");
}
public void testBug1987544() throws Exception {
testTypes(
"/** @param {string} x */ function foo(x) {}" +
"var duration;" +
"if (true && !(duration = 3)) {" +
" foo(duration);" +
"}",
"actual parameter 1 of foo does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testBug1940769() throws Exception {
testTypes(
"/** @return {!Object} */ " +
"function proto(obj) { return obj.prototype; }" +
"/** @constructor */ function Map() {}" +
"/**\n" +
" * @constructor\n" +
" * @extends {Map}\n" +
" */" +
"function Map2() { Map.call(this); };" +
"Map2.prototype = proto(Map);");
}
public void testBug2335992() throws Exception {
testTypes(
"/** @return {*} */ function f() { return 3; }" +
"var x = f();" +
"/** @type {string} */" +
"x.y = 3;",
"assignment\n" +
"found : number\n" +
"required: string");
}
public void testBug2341812() throws Exception {
testTypes(
"/** @interface */" +
"function EventTarget() {}" +
"/** @constructor \n * @implements {EventTarget} */" +
"function Node() {}" +
"/** @type {number} */ Node.prototype.index;" +
"/** @param {EventTarget} x \n * @return {string} */" +
"function foo(x) { return x.index; }");
}
public void testBug7701884() throws Exception {
testTypes(
"/**\n" +
" * @param {Array.<T>} x\n" +
" * @param {function(T)} y\n" +
" * @template T\n" +
" */\n" +
"var forEach = function(x, y) {\n" +
" for (var i = 0; i < x.length; i++) y(x[i]);\n" +
"};" +
"/** @param {number} x */" +
"function f(x) {}" +
"/** @param {?} x */" +
"function h(x) {" +
" var top = null;" +
" forEach(x, function(z) { top = z; });" +
" if (top) f(top);" +
"}");
}
public void testScopedConstructors1() throws Exception {
testTypes(
"function foo1() { " +
" /** @constructor */ function Bar() { " +
" /** @type {number} */ this.x = 3;" +
" }" +
"}" +
"function foo2() { " +
" /** @constructor */ function Bar() { " +
" /** @type {string} */ this.x = 'y';" +
" }" +
" /** " +
" * @param {Bar} b\n" +
" * @return {number}\n" +
" */" +
" function baz(b) { return b.x; }" +
"}",
"inconsistent return type\n" +
"found : string\n" +
"required: number");
}
public void testScopedConstructors2() throws Exception {
testTypes(
"/** @param {Function} f */" +
"function foo1(f) {" +
" /** @param {Function} g */" +
" f.prototype.bar = function(g) {};" +
"}");
}
public void testQualifiedNameInference1() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @type {number?} */ Foo.prototype.bar = null;" +
"/** @type {number?} */ Foo.prototype.baz = null;" +
"/** @param {Foo} foo */" +
"function f(foo) {" +
" while (true) {" +
" if (!foo.baz) break; " +
" foo.bar = null;" +
" }" +
// Tests a bug where this condition always evaluated to true.
" return foo.bar == null;" +
"}");
}
public void testQualifiedNameInference2() throws Exception {
testTypes(
"var x = {};" +
"x.y = c;" +
"function f(a, b) {" +
" if (a) {" +
" if (b) " +
" x.y = 2;" +
" else " +
" x.y = 1;" +
" }" +
" return x.y == null;" +
"}");
}
public void testQualifiedNameInference3() throws Exception {
testTypes(
"var x = {};" +
"x.y = c;" +
"function f(a, b) {" +
" if (a) {" +
" if (b) " +
" x.y = 2;" +
" else " +
" x.y = 1;" +
" }" +
" return x.y == null;" +
"} function g() { x.y = null; }");
}
public void testQualifiedNameInference4() throws Exception {
testTypes(
"/** @param {string} x */ function f(x) {}\n" +
"/**\n" +
" * @param {?string} x \n" +
" * @constructor\n" +
" */" +
"function Foo(x) { this.x_ = x; }\n" +
"Foo.prototype.bar = function() {" +
" if (this.x_) { f(this.x_); }" +
"};");
}
public void testQualifiedNameInference5() throws Exception {
testTypes(
"var ns = {}; " +
"(function() { " +
" /** @param {number} x */ ns.foo = function(x) {}; })();" +
"(function() { ns.foo(true); })();",
"actual parameter 1 of ns.foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testQualifiedNameInference6() throws Exception {
testTypes(
"/** @const */ var ns = {}; " +
"/** @param {number} x */ ns.foo = function(x) {};" +
"(function() { " +
" ns.foo = function(x) {};" +
" ns.foo(true); " +
"})();",
"actual parameter 1 of ns.foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testQualifiedNameInference7() throws Exception {
testTypes(
"var ns = {}; " +
"(function() { " +
" /** @constructor \n * @param {number} x */ " +
" ns.Foo = function(x) {};" +
" /** @param {ns.Foo} x */ function f(x) {}" +
" f(new ns.Foo(true));" +
"})();",
"actual parameter 1 of ns.Foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testQualifiedNameInference8() throws Exception {
// We may need to reshuffle name resolution order so that the @param
// type resolves correctly.
testClosureTypesMultipleWarnings(
"var ns = {}; " +
"(function() { " +
" /** @constructor \n * @param {number} x */ " +
" ns.Foo = function(x) {};" +
"})();" +
"/** @param {ns.Foo} x */ function f(x) {}" +
"f(new ns.Foo(true));",
Lists.newArrayList(
"Bad type annotation. Unknown type ns.Foo",
"actual parameter 1 of ns.Foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number"));
}
public void testQualifiedNameInference9() throws Exception {
testTypes(
"var ns = {}; " +
"ns.ns2 = {}; " +
"(function() { " +
" /** @constructor \n * @param {number} x */ " +
" ns.ns2.Foo = function(x) {};" +
" /** @param {ns.ns2.Foo} x */ function f(x) {}" +
" f(new ns.ns2.Foo(true));" +
"})();",
"actual parameter 1 of ns.ns2.Foo does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testQualifiedNameInference10() throws Exception {
testTypes(
"var ns = {}; " +
"ns.ns2 = {}; " +
"(function() { " +
" /** @interface */ " +
" ns.ns2.Foo = function() {};" +
" /** @constructor \n * @implements {ns.ns2.Foo} */ " +
" function F() {}" +
" (new F());" +
"})();");
}
public void testQualifiedNameInference11() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"function f() {" +
" var x = new Foo();" +
" x.onload = function() {" +
" x.onload = null;" +
" };" +
"}");
}
public void testQualifiedNameInference12() throws Exception {
// We should be able to tell that the two 'this' properties
// are different.
testTypes(
"/** @param {function(this:Object)} x */ function f(x) {}" +
"/** @constructor */ function Foo() {" +
" /** @type {number} */ this.bar = 3;" +
" f(function() { this.bar = true; });" +
"}");
}
public void testQualifiedNameInference13() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"function f(z) {" +
" var x = new Foo();" +
" if (z) {" +
" x.onload = function() {};" +
" } else {" +
" x.onload = null;" +
" };" +
"}");
}
public void testSheqRefinedScope() throws Exception {
Node n = parseAndTypeCheck(
"/** @constructor */function A() {}\n" +
"/** @constructor \n @extends A */ function B() {}\n" +
"/** @return {number} */\n" +
"B.prototype.p = function() { return 1; }\n" +
"/** @param {A} a\n @param {B} b */\n" +
"function f(a, b) {\n" +
" b.p();\n" +
" if (a === b) {\n" +
" b.p();\n" +
" }\n" +
"}");
Node nodeC = n.getLastChild().getLastChild().getLastChild().getLastChild()
.getLastChild().getLastChild();
JSType typeC = nodeC.getJSType();
assertTrue(typeC.isNumber());
Node nodeB = nodeC.getFirstChild().getFirstChild();
JSType typeB = nodeB.getJSType();
assertEquals("B", typeB.toString());
}
public void testAssignToUntypedVariable() throws Exception {
Node n = parseAndTypeCheck("var z; z = 1;");
Node assign = n.getLastChild().getFirstChild();
Node node = assign.getFirstChild();
assertFalse(node.getJSType().isUnknownType());
assertEquals("number", node.getJSType().toString());
}
public void testAssignToUntypedProperty() throws Exception {
Node n = parseAndTypeCheck(
"/** @constructor */ function Foo() {}\n" +
"Foo.prototype.a = 1;" +
"(new Foo).a;");
Node node = n.getLastChild().getFirstChild();
assertFalse(node.getJSType().isUnknownType());
assertTrue(node.getJSType().isNumber());
}
public void testNew1() throws Exception {
testTypes("new 4", TypeCheck.NOT_A_CONSTRUCTOR);
}
public void testNew2() throws Exception {
testTypes("var Math = {}; new Math()", TypeCheck.NOT_A_CONSTRUCTOR);
}
public void testNew3() throws Exception {
testTypes("new Date()");
}
public void testNew4() throws Exception {
testTypes("/** @constructor */function A(){}; new A();");
}
public void testNew5() throws Exception {
testTypes("function A(){}; new A();", TypeCheck.NOT_A_CONSTRUCTOR);
}
public void testNew6() throws Exception {
TypeCheckResult p =
parseAndTypeCheckWithScope("/** @constructor */function A(){};" +
"var a = new A();");
JSType aType = p.scope.getVar("a").getType();
assertTrue(aType instanceof ObjectType);
ObjectType aObjectType = (ObjectType) aType;
assertEquals("A", aObjectType.getConstructor().getReferenceName());
}
public void testNew7() throws Exception {
testTypes("/** @param {Function} opt_constructor */" +
"function foo(opt_constructor) {" +
"if (opt_constructor) { new opt_constructor; }" +
"}");
}
public void testNew8() throws Exception {
testTypes("/** @param {Function} opt_constructor */" +
"function foo(opt_constructor) {" +
"new opt_constructor;" +
"}");
}
public void testNew9() throws Exception {
testTypes("/** @param {Function} opt_constructor */" +
"function foo(opt_constructor) {" +
"new (opt_constructor || Array);" +
"}");
}
public void testNew10() throws Exception {
testTypes("var goog = {};" +
"/** @param {Function} opt_constructor */" +
"goog.Foo = function (opt_constructor) {" +
"new (opt_constructor || Array);" +
"}");
}
public void testNew11() throws Exception {
testTypes("/** @param {Function} c1 */" +
"function f(c1) {" +
" var c2 = function(){};" +
" c1.prototype = new c2;" +
"}", TypeCheck.NOT_A_CONSTRUCTOR);
}
public void testNew12() throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope("var a = new Array();");
Var a = p.scope.getVar("a");
assertTypeEquals(ARRAY_TYPE, a.getType());
}
public void testNew13() throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope(
"/** @constructor */function FooBar(){};" +
"var a = new FooBar();");
Var a = p.scope.getVar("a");
assertTrue(a.getType() instanceof ObjectType);
assertEquals("FooBar", a.getType().toString());
}
public void testNew14() throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope(
"/** @constructor */var FooBar = function(){};" +
"var a = new FooBar();");
Var a = p.scope.getVar("a");
assertTrue(a.getType() instanceof ObjectType);
assertEquals("FooBar", a.getType().toString());
}
public void testNew15() throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope(
"var goog = {};" +
"/** @constructor */goog.A = function(){};" +
"var a = new goog.A();");
Var a = p.scope.getVar("a");
assertTrue(a.getType() instanceof ObjectType);
assertEquals("goog.A", a.getType().toString());
}
public void testNew16() throws Exception {
testTypes(
"/** \n" +
" * @param {string} x \n" +
" * @constructor \n" +
" */" +
"function Foo(x) {}" +
"function g() { new Foo(1); }",
"actual parameter 1 of Foo does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testNew17() throws Exception {
testTypes("var goog = {}; goog.x = 3; new goog.x",
"cannot instantiate non-constructor");
}
public void testNew18() throws Exception {
testTypes("var goog = {};" +
"/** @constructor */ goog.F = function() {};" +
"/** @constructor */ goog.G = goog.F;");
}
public void testName1() throws Exception {
assertTypeEquals(VOID_TYPE, testNameNode("undefined"));
}
public void testName2() throws Exception {
assertTypeEquals(OBJECT_FUNCTION_TYPE, testNameNode("Object"));
}
public void testName3() throws Exception {
assertTypeEquals(ARRAY_FUNCTION_TYPE, testNameNode("Array"));
}
public void testName4() throws Exception {
assertTypeEquals(DATE_FUNCTION_TYPE, testNameNode("Date"));
}
public void testName5() throws Exception {
assertTypeEquals(REGEXP_FUNCTION_TYPE, testNameNode("RegExp"));
}
/**
* Type checks a NAME node and retrieve its type.
*/
private JSType testNameNode(String name) {
Node node = Node.newString(Token.NAME, name);
Node parent = new Node(Token.SCRIPT, node);
parent.setInputId(new InputId("code"));
Node externs = new Node(Token.SCRIPT);
externs.setInputId(new InputId("externs"));
Node externAndJsRoot = new Node(Token.BLOCK, externs, parent);
externAndJsRoot.setIsSyntheticBlock(true);
makeTypeCheck().processForTesting(null, parent);
return node.getJSType();
}
public void testBitOperation1() throws Exception {
testTypes("/**@return {void}*/function foo(){ ~foo(); }",
"operator ~ cannot be applied to undefined");
}
public void testBitOperation2() throws Exception {
testTypes("/**@return {void}*/function foo(){var a = foo()<<3;}",
"operator << cannot be applied to undefined");
}
public void testBitOperation3() throws Exception {
testTypes("/**@return {void}*/function foo(){var a = 3<<foo();}",
"operator << cannot be applied to undefined");
}
public void testBitOperation4() throws Exception {
testTypes("/**@return {void}*/function foo(){var a = foo()>>>3;}",
"operator >>> cannot be applied to undefined");
}
public void testBitOperation5() throws Exception {
testTypes("/**@return {void}*/function foo(){var a = 3>>>foo();}",
"operator >>> cannot be applied to undefined");
}
public void testBitOperation6() throws Exception {
testTypes("/**@return {!Object}*/function foo(){var a = foo()&3;}",
"bad left operand to bitwise operator\n" +
"found : Object\n" +
"required: (boolean|null|number|string|undefined)");
}
public void testBitOperation7() throws Exception {
testTypes("var x = null; x |= undefined; x &= 3; x ^= '3'; x |= true;");
}
public void testBitOperation8() throws Exception {
testTypes("var x = void 0; x |= new Number(3);");
}
public void testBitOperation9() throws Exception {
testTypes("var x = void 0; x |= {};",
"bad right operand to bitwise operator\n" +
"found : {}\n" +
"required: (boolean|null|number|string|undefined)");
}
public void testCall1() throws Exception {
testTypes("3();", "number expressions are not callable");
}
public void testCall2() throws Exception {
testTypes("/** @param {!Number} foo*/function bar(foo){ bar('abc'); }",
"actual parameter 1 of bar does not match formal parameter\n" +
"found : string\n" +
"required: Number");
}
public void testCall3() throws Exception {
// We are checking that an unresolved named type can successfully
// meet with a functional type to produce a callable type.
testTypes("/** @type {Function|undefined} */var opt_f;" +
"/** @type {some.unknown.type} */var f1;" +
"var f2 = opt_f || f1;" +
"f2();",
"Bad type annotation. Unknown type some.unknown.type");
}
public void testCall4() throws Exception {
testTypes("/**@param {!RegExp} a*/var foo = function bar(a){ bar('abc'); }",
"actual parameter 1 of bar does not match formal parameter\n" +
"found : string\n" +
"required: RegExp");
}
public void testCall5() throws Exception {
testTypes("/**@param {!RegExp} a*/var foo = function bar(a){ foo('abc'); }",
"actual parameter 1 of foo does not match formal parameter\n" +
"found : string\n" +
"required: RegExp");
}
public void testCall6() throws Exception {
testTypes("/** @param {!Number} foo*/function bar(foo){}" +
"bar('abc');",
"actual parameter 1 of bar does not match formal parameter\n" +
"found : string\n" +
"required: Number");
}
public void testCall7() throws Exception {
testTypes("/** @param {!RegExp} a*/var foo = function bar(a){};" +
"foo('abc');",
"actual parameter 1 of foo does not match formal parameter\n" +
"found : string\n" +
"required: RegExp");
}
public void testCall8() throws Exception {
testTypes("/** @type {Function|number} */var f;f();",
"(Function|number) expressions are " +
"not callable");
}
public void testCall9() throws Exception {
testTypes(
"var goog = {};" +
"/** @constructor */ goog.Foo = function() {};" +
"/** @param {!goog.Foo} a */ var bar = function(a){};" +
"bar('abc');",
"actual parameter 1 of bar does not match formal parameter\n" +
"found : string\n" +
"required: goog.Foo");
}
public void testCall10() throws Exception {
testTypes("/** @type {Function} */var f;f();");
}
public void testCall11() throws Exception {
testTypes("var f = new Function(); f();");
}
public void testFunctionCall1() throws Exception {
testTypes(
"/** @param {number} x */ var foo = function(x) {};" +
"foo.call(null, 3);");
}
public void testFunctionCall2() throws Exception {
testTypes(
"/** @param {number} x */ var foo = function(x) {};" +
"foo.call(null, 'bar');",
"actual parameter 2 of foo.call does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testFunctionCall3() throws Exception {
testTypes(
"/** @param {number} x \n * @constructor */ " +
"var Foo = function(x) { this.bar.call(null, x); };" +
"/** @type {function(number)} */ Foo.prototype.bar;");
}
public void testFunctionCall4() throws Exception {
testTypes(
"/** @param {string} x \n * @constructor */ " +
"var Foo = function(x) { this.bar.call(null, x); };" +
"/** @type {function(number)} */ Foo.prototype.bar;",
"actual parameter 2 of this.bar.call " +
"does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testFunctionCall5() throws Exception {
testTypes(
"/** @param {Function} handler \n * @constructor */ " +
"var Foo = function(handler) { handler.call(this, x); };");
}
public void testFunctionCall6() throws Exception {
testTypes(
"/** @param {Function} handler \n * @constructor */ " +
"var Foo = function(handler) { handler.apply(this, x); };");
}
public void testFunctionCall7() throws Exception {
testTypes(
"/** @param {Function} handler \n * @param {Object} opt_context */ " +
"var Foo = function(handler, opt_context) { " +
" handler.call(opt_context, x);" +
"};");
}
public void testFunctionCall8() throws Exception {
testTypes(
"/** @param {Function} handler \n * @param {Object} opt_context */ " +
"var Foo = function(handler, opt_context) { " +
" handler.apply(opt_context, x);" +
"};");
}
public void testFunctionBind1() throws Exception {
testTypes(
"/** @type {function(string, number): boolean} */" +
"function f(x, y) { return true; }" +
"f.bind(null, 3);",
"actual parameter 2 of f.bind does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testFunctionBind2() throws Exception {
testTypes(
"/** @type {function(number): boolean} */" +
"function f(x) { return true; }" +
"f(f.bind(null, 3)());",
"actual parameter 1 of f does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testFunctionBind3() throws Exception {
testTypes(
"/** @type {function(number, string): boolean} */" +
"function f(x, y) { return true; }" +
"f.bind(null, 3)(true);",
"actual parameter 1 of function does not match formal parameter\n" +
"found : boolean\n" +
"required: string");
}
public void testFunctionBind4() throws Exception {
testTypes(
"/** @param {...number} x */" +
"function f(x) {}" +
"f.bind(null, 3, 3, 3)(true);",
"actual parameter 1 of function does not match formal parameter\n" +
"found : boolean\n" +
"required: (number|undefined)");
}
public void testFunctionBind5() throws Exception {
testTypes(
"/** @param {...number} x */" +
"function f(x) {}" +
"f.bind(null, true)(3, 3, 3);",
"actual parameter 2 of f.bind does not match formal parameter\n" +
"found : boolean\n" +
"required: (number|undefined)");
}
public void testGoogBind1() throws Exception {
testClosureTypes(
"var goog = {}; goog.bind = function(var_args) {};" +
"/** @type {function(number): boolean} */" +
"function f(x, y) { return true; }" +
"f(goog.bind(f, null, 'x')());",
"actual parameter 1 of f does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testGoogBind2() throws Exception {
// TODO(nicksantos): We do not currently type-check the arguments
// of the goog.bind.
testClosureTypes(
"var goog = {}; goog.bind = function(var_args) {};" +
"/** @type {function(boolean): boolean} */" +
"function f(x, y) { return true; }" +
"f(goog.bind(f, null, 'x')());",
null);
}
public void testCast2() throws Exception {
// can upcast to a base type.
testTypes("/** @constructor */function base() {}\n" +
"/** @constructor\n @extends {base} */function derived() {}\n" +
"/** @type {base} */ var baz = new derived();\n");
}
public void testCast3() throws Exception {
// cannot downcast
testTypes("/** @constructor */function base() {}\n" +
"/** @constructor @extends {base} */function derived() {}\n" +
"/** @type {!derived} */ var baz = new base();\n",
"initializing variable\n" +
"found : base\n" +
"required: derived");
}
public void testCast3a() throws Exception {
// cannot downcast
testTypes("/** @constructor */function Base() {}\n" +
"/** @constructor @extends {Base} */function Derived() {}\n" +
"var baseInstance = new Base();" +
"/** @type {!Derived} */ var baz = baseInstance;\n",
"initializing variable\n" +
"found : Base\n" +
"required: Derived");
}
public void testCast4() throws Exception {
// downcast must be explicit
testTypes("/** @constructor */function base() {}\n" +
"/** @constructor\n * @extends {base} */function derived() {}\n" +
"/** @type {!derived} */ var baz = " +
"/** @type {!derived} */(new base());\n");
}
public void testCast5() throws Exception {
// cannot explicitly cast to an unrelated type
testTypes("/** @constructor */function foo() {}\n" +
"/** @constructor */function bar() {}\n" +
"var baz = /** @type {!foo} */(new bar);\n",
"invalid cast - must be a subtype or supertype\n" +
"from: bar\n" +
"to : foo");
}
public void testCast5a() throws Exception {
// cannot explicitly cast to an unrelated type
testTypes("/** @constructor */function foo() {}\n" +
"/** @constructor */function bar() {}\n" +
"var barInstance = new bar;\n" +
"var baz = /** @type {!foo} */(barInstance);\n",
"invalid cast - must be a subtype or supertype\n" +
"from: bar\n" +
"to : foo");
}
public void testCast6() throws Exception {
// can explicitly cast to a subtype or supertype
testTypes("/** @constructor */function foo() {}\n" +
"/** @constructor \n @extends foo */function bar() {}\n" +
"var baz = /** @type {!bar} */(new bar);\n" +
"var baz = /** @type {!foo} */(new foo);\n" +
"var baz = /** @type {bar} */(new bar);\n" +
"var baz = /** @type {foo} */(new foo);\n" +
"var baz = /** @type {!foo} */(new bar);\n" +
"var baz = /** @type {!bar} */(new foo);\n" +
"var baz = /** @type {foo} */(new bar);\n" +
"var baz = /** @type {bar} */(new foo);\n");
}
public void testCast7() throws Exception {
testTypes("var x = /** @type {foo} */ (new Object());",
"Bad type annotation. Unknown type foo");
}
public void testCast8() throws Exception {
testTypes("function f() { return /** @type {foo} */ (new Object()); }",
"Bad type annotation. Unknown type foo");
}
public void testCast9() throws Exception {
testTypes("var foo = {};" +
"function f() { return /** @type {foo} */ (new Object()); }",
"Bad type annotation. Unknown type foo");
}
public void testCast10() throws Exception {
testTypes("var foo = function() {};" +
"function f() { return /** @type {foo} */ (new Object()); }",
"Bad type annotation. Unknown type foo");
}
public void testCast11() throws Exception {
testTypes("var goog = {}; goog.foo = {};" +
"function f() { return /** @type {goog.foo} */ (new Object()); }",
"Bad type annotation. Unknown type goog.foo");
}
public void testCast12() throws Exception {
testTypes("var goog = {}; goog.foo = function() {};" +
"function f() { return /** @type {goog.foo} */ (new Object()); }",
"Bad type annotation. Unknown type goog.foo");
}
public void testCast13() throws Exception {
// Test to make sure that the forward-declaration still allows for
// a warning.
testClosureTypes("var goog = {}; " +
"goog.addDependency('zzz.js', ['goog.foo'], []);" +
"goog.foo = function() {};" +
"function f() { return /** @type {goog.foo} */ (new Object()); }",
"Bad type annotation. Unknown type goog.foo");
}
public void testCast14() throws Exception {
// Test to make sure that the forward-declaration still prevents
// some warnings.
testClosureTypes("var goog = {}; " +
"goog.addDependency('zzz.js', ['goog.bar'], []);" +
"function f() { return /** @type {goog.bar} */ (new Object()); }",
null);
}
public void testCast15() throws Exception {
// This fixes a bug where a type cast on an object literal
// would cause a run-time cast exception if the node was visited
// more than once.
//
// Some code assumes that an object literal must have a object type,
// while because of the cast, it could have any type (including
// a union).
testTypes(
"for (var i = 0; i < 10; i++) {" +
"var x = /** @type {Object|number} */ ({foo: 3});" +
"/** @param {number} x */ function f(x) {}" +
"f(x.foo);" +
"f([].foo);" +
"}",
"Property foo never defined on Array");
}
public void testCast16() throws Exception {
// A type cast should not invalidate the checks on the members
testTypes(
"for (var i = 0; i < 10; i++) {" +
"var x = /** @type {Object|number} */ (" +
" {/** @type {string} */ foo: 3});" +
"}",
"assignment to property foo of Object\n" +
"found : number\n" +
"required: string");
}
public void testCast17a() throws Exception {
// Mostly verifying that rhino actually understands these JsDocs.
testTypes("/** @constructor */ function Foo() {} \n" +
"/** @type {Foo} */ var x = /** @type {Foo} */ (y)");
testTypes("/** @constructor */ function Foo() {} \n" +
"/** @type {Foo} */ var x = (/** @type {Foo} */ y)");
}
public void testCast17b() throws Exception {
// Mostly verifying that rhino actually understands these JsDocs.
testTypes("/** @constructor */ function Foo() {} \n" +
"/** @type {Foo} */ var x = /** @type {Foo} */ ({})");
}
public void testCast18() throws Exception {
// Mostly verifying that legacy annotations are applied
// despite the parser warning.
testTypes("/** @constructor */ function Foo() {} \n" +
"/** @type {Foo} */ var x = (/** @type {Foo} */ {})",
"Type annotations are not allowed here. " +
"Are you missing parentheses?");
// Not really encourage because of possible ambiguity but it works.
testTypes("/** @constructor */ function Foo() {} \n" +
"/** @type {Foo} */ var x = /** @type {Foo} */ {}",
"Type annotations are not allowed here. " +
"Are you missing parentheses?");
}
public void testCast19() throws Exception {
testTypes(
"var x = 'string';\n" +
"/** @type {number} */\n" +
"var y = /** @type {number} */(x);",
"invalid cast - must be a subtype or supertype\n" +
"from: string\n" +
"to : number");
}
public void testCast20() throws Exception {
testTypes(
"/** @enum {boolean|null} */\n" +
"var X = {" +
" AA: true," +
" BB: false," +
" CC: null" +
"};\n" +
"var y = /** @type {X} */(true);");
}
public void testCast21() throws Exception {
testTypes(
"/** @enum {boolean|null} */\n" +
"var X = {" +
" AA: true," +
" BB: false," +
" CC: null" +
"};\n" +
"var value = true;\n" +
"var y = /** @type {X} */(value);");
}
public void testCast22() throws Exception {
testTypes(
"var x = null;\n" +
"var y = /** @type {number} */(x);",
"invalid cast - must be a subtype or supertype\n" +
"from: null\n" +
"to : number");
}
public void testCast23() throws Exception {
testTypes(
"var x = null;\n" +
"var y = /** @type {Number} */(x);");
}
public void testCast24() throws Exception {
testTypes(
"var x = undefined;\n" +
"var y = /** @type {number} */(x);",
"invalid cast - must be a subtype or supertype\n" +
"from: undefined\n" +
"to : number");
}
public void testCast25() throws Exception {
testTypes(
"var x = undefined;\n" +
"var y = /** @type {number|undefined} */(x);");
}
public void testCast26() throws Exception {
testTypes(
"function fn(dir) {\n" +
" var node = dir ? 1 : 2;\n" +
" fn(/** @type {number} */ (node));\n" +
"}");
}
public void testCast27() throws Exception {
// C doesn't implement I but a subtype might.
testTypes(
"/** @interface */ function I() {}\n" +
"/** @constructor */ function C() {}\n" +
"var x = new C();\n" +
"var y = /** @type {I} */(x);");
}
public void testCast27a() throws Exception {
// C doesn't implement I but a subtype might.
testTypes(
"/** @interface */ function I() {}\n" +
"/** @constructor */ function C() {}\n" +
"/** @type {C} */ var x ;\n" +
"var y = /** @type {I} */(x);");
}
public void testCast28() throws Exception {
// C doesn't implement I but a subtype might.
testTypes(
"/** @interface */ function I() {}\n" +
"/** @constructor */ function C() {}\n" +
"/** @type {!I} */ var x;\n" +
"var y = /** @type {C} */(x);");
}
public void testCast28a() throws Exception {
// C doesn't implement I but a subtype might.
testTypes(
"/** @interface */ function I() {}\n" +
"/** @constructor */ function C() {}\n" +
"/** @type {I} */ var x;\n" +
"var y = /** @type {C} */(x);");
}
public void testCast29a() throws Exception {
// C doesn't implement the record type but a subtype might.
testTypes(
"/** @constructor */ function C() {}\n" +
"var x = new C();\n" +
"var y = /** @type {{remoteJids: Array, sessionId: string}} */(x);");
}
public void testCast29b() throws Exception {
// C doesn't implement the record type but a subtype might.
testTypes(
"/** @constructor */ function C() {}\n" +
"/** @type {C} */ var x;\n" +
"var y = /** @type {{prop1: Array, prop2: string}} */(x);");
}
public void testCast29c() throws Exception {
// C doesn't implement the record type but a subtype might.
testTypes(
"/** @constructor */ function C() {}\n" +
"/** @type {{remoteJids: Array, sessionId: string}} */ var x ;\n" +
"var y = /** @type {C} */(x);");
}
public void testCast30() throws Exception {
// Should be able to cast to a looser return type
testTypes(
"/** @constructor */ function C() {}\n" +
"/** @type {function():string} */ var x ;\n" +
"var y = /** @type {function():?} */(x);");
}
public void testCast31() throws Exception {
// Should be able to cast to a tighter parameter type
testTypes(
"/** @constructor */ function C() {}\n" +
"/** @type {function(*)} */ var x ;\n" +
"var y = /** @type {function(string)} */(x);");
}
public void testCast32() throws Exception {
testTypes(
"/** @constructor */ function C() {}\n" +
"/** @type {Object} */ var x ;\n" +
"var y = /** @type {null|{length:number}} */(x);");
}
public void testCast33() throws Exception {
// null and void should be assignable to any type that accepts one or the
// other or both.
testTypes(
"/** @constructor */ function C() {}\n" +
"/** @type {null|undefined} */ var x ;\n" +
"var y = /** @type {string?|undefined} */(x);");
testTypes(
"/** @constructor */ function C() {}\n" +
"/** @type {null|undefined} */ var x ;\n" +
"var y = /** @type {string|undefined} */(x);");
testTypes(
"/** @constructor */ function C() {}\n" +
"/** @type {null|undefined} */ var x ;\n" +
"var y = /** @type {string?} */(x);");
testTypes(
"/** @constructor */ function C() {}\n" +
"/** @type {null|undefined} */ var x ;\n" +
"var y = /** @type {null} */(x);");
}
public void testCast34a() throws Exception {
testTypes(
"/** @constructor */ function C() {}\n" +
"/** @type {Object} */ var x ;\n" +
"var y = /** @type {Function} */(x);");
}
public void testCast34b() throws Exception {
testTypes(
"/** @constructor */ function C() {}\n" +
"/** @type {Function} */ var x ;\n" +
"var y = /** @type {Object} */(x);");
}
public void testNestedCasts() throws Exception {
testTypes("/** @constructor */var T = function() {};\n" +
"/** @constructor */var V = function() {};\n" +
"/**\n" +
"* @param {boolean} b\n" +
"* @return {T|V}\n" +
"*/\n" +
"function f(b) { return b ? new T() : new V(); }\n" +
"/**\n" +
"* @param {boolean} b\n" +
"* @return {boolean|undefined}\n" +
"*/\n" +
"function g(b) { return b ? true : undefined; }\n" +
"/** @return {T} */\n" +
"function h() {\n" +
"return /** @type {T} */ (f(/** @type {boolean} */ (g(true))));\n" +
"}");
}
public void testNativeCast1() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"f(String(true));",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testNativeCast2() throws Exception {
testTypes(
"/** @param {string} x */ function f(x) {}" +
"f(Number(true));",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testNativeCast3() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"f(Boolean(''));",
"actual parameter 1 of f does not match formal parameter\n" +
"found : boolean\n" +
"required: number");
}
public void testNativeCast4() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"f(Error(''));",
"actual parameter 1 of f does not match formal parameter\n" +
"found : Error\n" +
"required: number");
}
public void testBadConstructorCall() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo();",
"Constructor function (new:Foo): undefined should be called " +
"with the \"new\" keyword");
}
public void testTypeof() throws Exception {
testTypes("/**@return {void}*/function foo(){ var a = typeof foo(); }");
}
public void testTypeof2() throws Exception {
testTypes("function f(){ if (typeof 123 == 'numbr') return 321; }",
"unknown type: numbr");
}
public void testTypeof3() throws Exception {
testTypes("function f() {" +
"return (typeof 123 == 'number' ||" +
"typeof 123 == 'string' ||" +
"typeof 123 == 'boolean' ||" +
"typeof 123 == 'undefined' ||" +
"typeof 123 == 'function' ||" +
"typeof 123 == 'object' ||" +
"typeof 123 == 'unknown'); }");
}
public void testConstructorType1() throws Exception {
testTypes("/**@constructor*/function Foo(){}" +
"/**@type{!Foo}*/var f = new Date();",
"initializing variable\n" +
"found : Date\n" +
"required: Foo");
}
public void testConstructorType2() throws Exception {
testTypes("/**@constructor*/function Foo(){\n" +
"/**@type{Number}*/this.bar = new Number(5);\n" +
"}\n" +
"/**@type{Foo}*/var f = new Foo();\n" +
"/**@type{Number}*/var n = f.bar;");
}
public void testConstructorType3() throws Exception {
// Reverse the declaration order so that we know that Foo is getting set
// even on an out-of-order declaration sequence.
testTypes("/**@type{Foo}*/var f = new Foo();\n" +
"/**@type{Number}*/var n = f.bar;" +
"/**@constructor*/function Foo(){\n" +
"/**@type{Number}*/this.bar = new Number(5);\n" +
"}\n");
}
public void testConstructorType4() throws Exception {
testTypes("/**@constructor*/function Foo(){\n" +
"/**@type{!Number}*/this.bar = new Number(5);\n" +
"}\n" +
"/**@type{!Foo}*/var f = new Foo();\n" +
"/**@type{!String}*/var n = f.bar;",
"initializing variable\n" +
"found : Number\n" +
"required: String");
}
public void testConstructorType5() throws Exception {
testTypes("/**@constructor*/function Foo(){}\n" +
"if (Foo){}\n");
}
public void testConstructorType6() throws Exception {
testTypes("/** @constructor */\n" +
"function bar() {}\n" +
"function _foo() {\n" +
" /** @param {bar} x */\n" +
" function f(x) {}\n" +
"}");
}
public void testConstructorType7() throws Exception {
TypeCheckResult p =
parseAndTypeCheckWithScope("/** @constructor */function A(){};");
JSType type = p.scope.getVar("A").getType();
assertTrue(type instanceof FunctionType);
FunctionType fType = (FunctionType) type;
assertEquals("A", fType.getReferenceName());
}
public void testConstructorType8() throws Exception {
testTypes(
"var ns = {};" +
"ns.create = function() { return function() {}; };" +
"/** @constructor */ ns.Foo = ns.create();" +
"ns.Foo.prototype = {x: 0, y: 0};" +
"/**\n" +
" * @param {ns.Foo} foo\n" +
" * @return {string}\n" +
" */\n" +
"function f(foo) {" +
" return foo.x;" +
"}",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testConstructorType9() throws Exception {
testTypes(
"var ns = {};" +
"ns.create = function() { return function() {}; };" +
"ns.extend = function(x) { return x; };" +
"/** @constructor */ ns.Foo = ns.create();" +
"ns.Foo.prototype = ns.extend({x: 0, y: 0});" +
"/**\n" +
" * @param {ns.Foo} foo\n" +
" * @return {string}\n" +
" */\n" +
"function f(foo) {" +
" return foo.x;" +
"}");
}
public void testConstructorType10() throws Exception {
testTypes("/** @constructor */" +
"function NonStr() {}" +
"/**\n" +
" * @constructor\n" +
" * @struct\n" +
" * @extends{NonStr}\n" +
" */" +
"function NonStrKid() {}",
"NonStrKid cannot extend this type; " +
"structs can only extend structs");
}
public void testConstructorType11() throws Exception {
testTypes("/** @constructor */" +
"function NonDict() {}" +
"/**\n" +
" * @constructor\n" +
" * @dict\n" +
" * @extends{NonDict}\n" +
" */" +
"function NonDictKid() {}",
"NonDictKid cannot extend this type; " +
"dicts can only extend dicts");
}
public void testConstructorType12() throws Exception {
testTypes("/**\n" +
" * @constructor\n" +
" * @struct\n" +
" */\n" +
"function Bar() {}\n" +
"Bar.prototype = {};\n",
"Bar cannot extend this type; " +
"structs can only extend structs");
}
public void testBadStruct() throws Exception {
testTypes("/** @struct */function Struct1() {}",
"@struct used without @constructor for Struct1");
}
public void testBadDict() throws Exception {
testTypes("/** @dict */function Dict1() {}",
"@dict used without @constructor for Dict1");
}
public void testAnonymousPrototype1() throws Exception {
testTypes(
"var ns = {};" +
"/** @constructor */ ns.Foo = function() {" +
" this.bar(3, 5);" +
"};" +
"ns.Foo.prototype = {" +
" bar: function(x) {}" +
"};",
"Function ns.Foo.prototype.bar: called with 2 argument(s). " +
"Function requires at least 1 argument(s) and no more " +
"than 1 argument(s).");
}
public void testAnonymousPrototype2() throws Exception {
testTypes(
"/** @interface */ var Foo = function() {};" +
"Foo.prototype = {" +
" foo: function(x) {}" +
"};" +
"/**\n" +
" * @constructor\n" +
" * @implements {Foo}\n" +
" */ var Bar = function() {};",
"property foo on interface Foo is not implemented by type Bar");
}
public void testAnonymousType1() throws Exception {
testTypes("function f() { return {}; }" +
"/** @constructor */\n" +
"f().bar = function() {};");
}
public void testAnonymousType2() throws Exception {
testTypes("function f() { return {}; }" +
"/** @interface */\n" +
"f().bar = function() {};");
}
public void testAnonymousType3() throws Exception {
testTypes("function f() { return {}; }" +
"/** @enum */\n" +
"f().bar = {FOO: 1};");
}
public void testBang1() throws Exception {
testTypes("/** @param {Object} x\n@return {!Object} */\n" +
"function f(x) { return x; }",
"inconsistent return type\n" +
"found : (Object|null)\n" +
"required: Object");
}
public void testBang2() throws Exception {
testTypes("/** @param {Object} x\n@return {!Object} */\n" +
"function f(x) { return x ? x : new Object(); }");
}
public void testBang3() throws Exception {
testTypes("/** @param {Object} x\n@return {!Object} */\n" +
"function f(x) { return /** @type {!Object} */ (x); }");
}
public void testBang4() throws Exception {
testTypes("/**@param {Object} x\n@param {Object} y\n@return {boolean}*/\n" +
"function f(x, y) {\n" +
"if (typeof x != 'undefined') { return x == y; }\n" +
"else { return x != y; }\n}");
}
public void testBang5() throws Exception {
testTypes("/**@param {Object} x\n@param {Object} y\n@return {boolean}*/\n" +
"function f(x, y) { return !!x && x == y; }");
}
public void testBang6() throws Exception {
testTypes("/** @param {Object?} x\n@return {Object} */\n" +
"function f(x) { return x; }");
}
public void testBang7() throws Exception {
testTypes("/**@param {(Object,string,null)} x\n" +
"@return {(Object,string)}*/function f(x) { return x; }");
}
public void testDefinePropertyOnNullableObject1() throws Exception {
testTypes("/** @type {Object} */ var n = {};\n" +
"/** @type {number} */ n.x = 1;\n" +
"/** @return {boolean} */function f() { return n.x; }",
"inconsistent return type\n" +
"found : number\n" +
"required: boolean");
}
public void testDefinePropertyOnNullableObject2() throws Exception {
testTypes("/** @constructor */ var T = function() {};\n" +
"/** @param {T} t\n@return {boolean} */function f(t) {\n" +
"t.x = 1; return t.x; }",
"inconsistent return type\n" +
"found : number\n" +
"required: boolean");
}
public void testUnknownConstructorInstanceType1() throws Exception {
testTypes("/** @return {Array} */ function g(f) { return new f(); }");
}
public void testUnknownConstructorInstanceType2() throws Exception {
testTypes("function g(f) { return /** @type Array */(new f()); }");
}
public void testUnknownConstructorInstanceType3() throws Exception {
testTypes("function g(f) { var x = new f(); x.a = 1; return x; }");
}
public void testUnknownPrototypeChain() throws Exception {
testTypes("/**\n" +
"* @param {Object} co\n" +
" * @return {Object}\n" +
" */\n" +
"function inst(co) {\n" +
" /** @constructor */\n" +
" var c = function() {};\n" +
" c.prototype = co.prototype;\n" +
" return new c;\n" +
"}");
}
public void testNamespacedConstructor() throws Exception {
Node root = parseAndTypeCheck(
"var goog = {};" +
"/** @constructor */ goog.MyClass = function() {};" +
"/** @return {!goog.MyClass} */ " +
"function foo() { return new goog.MyClass(); }");
JSType typeOfFoo = root.getLastChild().getJSType();
assert(typeOfFoo instanceof FunctionType);
JSType retType = ((FunctionType) typeOfFoo).getReturnType();
assert(retType instanceof ObjectType);
assertEquals("goog.MyClass", ((ObjectType) retType).getReferenceName());
}
public void testComplexNamespace() throws Exception {
String js =
"var goog = {};" +
"goog.foo = {};" +
"goog.foo.bar = 5;";
TypeCheckResult p = parseAndTypeCheckWithScope(js);
// goog type in the scope
JSType googScopeType = p.scope.getVar("goog").getType();
assertTrue(googScopeType instanceof ObjectType);
assertTrue("foo property not present on goog type",
((ObjectType) googScopeType).hasProperty("foo"));
assertFalse("bar property present on goog type",
((ObjectType) googScopeType).hasProperty("bar"));
// goog type on the VAR node
Node varNode = p.root.getFirstChild();
assertEquals(Token.VAR, varNode.getType());
JSType googNodeType = varNode.getFirstChild().getJSType();
assertTrue(googNodeType instanceof ObjectType);
// goog scope type and goog type on VAR node must be the same
assertTrue(googScopeType == googNodeType);
// goog type on the left of the GETPROP node (under fist ASSIGN)
Node getpropFoo1 = varNode.getNext().getFirstChild().getFirstChild();
assertEquals(Token.GETPROP, getpropFoo1.getType());
assertEquals("goog", getpropFoo1.getFirstChild().getString());
JSType googGetpropFoo1Type = getpropFoo1.getFirstChild().getJSType();
assertTrue(googGetpropFoo1Type instanceof ObjectType);
// still the same type as the one on the variable
assertTrue(googGetpropFoo1Type == googScopeType);
// the foo property should be defined on goog
JSType googFooType = ((ObjectType) googScopeType).getPropertyType("foo");
assertTrue(googFooType instanceof ObjectType);
// goog type on the left of the GETPROP lower level node
// (under second ASSIGN)
Node getpropFoo2 = varNode.getNext().getNext()
.getFirstChild().getFirstChild().getFirstChild();
assertEquals(Token.GETPROP, getpropFoo2.getType());
assertEquals("goog", getpropFoo2.getFirstChild().getString());
JSType googGetpropFoo2Type = getpropFoo2.getFirstChild().getJSType();
assertTrue(googGetpropFoo2Type instanceof ObjectType);
// still the same type as the one on the variable
assertTrue(googGetpropFoo2Type == googScopeType);
// goog.foo type on the left of the top-level GETPROP node
// (under second ASSIGN)
JSType googFooGetprop2Type = getpropFoo2.getJSType();
assertTrue("goog.foo incorrectly annotated in goog.foo.bar selection",
googFooGetprop2Type instanceof ObjectType);
ObjectType googFooGetprop2ObjectType = (ObjectType) googFooGetprop2Type;
assertFalse("foo property present on goog.foo type",
googFooGetprop2ObjectType.hasProperty("foo"));
assertTrue("bar property not present on goog.foo type",
googFooGetprop2ObjectType.hasProperty("bar"));
assertTypeEquals("bar property on goog.foo type incorrectly inferred",
NUMBER_TYPE, googFooGetprop2ObjectType.getPropertyType("bar"));
}
public void testAddingMethodsUsingPrototypeIdiomSimpleNamespace()
throws Exception {
Node js1Node = parseAndTypeCheck(
"/** @constructor */function A() {}" +
"A.prototype.m1 = 5");
ObjectType instanceType = getInstanceType(js1Node);
assertEquals(NATIVE_PROPERTIES_COUNT + 1,
instanceType.getPropertiesCount());
checkObjectType(instanceType, "m1", NUMBER_TYPE);
}
public void testAddingMethodsUsingPrototypeIdiomComplexNamespace1()
throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope(
"var goog = {};" +
"goog.A = /** @constructor */function() {};" +
"/** @type number */goog.A.prototype.m1 = 5");
testAddingMethodsUsingPrototypeIdiomComplexNamespace(p);
}
public void testAddingMethodsUsingPrototypeIdiomComplexNamespace2()
throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope(
"var goog = {};" +
"/** @constructor */goog.A = function() {};" +
"/** @type number */goog.A.prototype.m1 = 5");
testAddingMethodsUsingPrototypeIdiomComplexNamespace(p);
}
private void testAddingMethodsUsingPrototypeIdiomComplexNamespace(
TypeCheckResult p) {
ObjectType goog = (ObjectType) p.scope.getVar("goog").getType();
assertEquals(NATIVE_PROPERTIES_COUNT + 1, goog.getPropertiesCount());
JSType googA = goog.getPropertyType("A");
assertNotNull(googA);
assertTrue(googA instanceof FunctionType);
FunctionType googAFunction = (FunctionType) googA;
ObjectType classA = googAFunction.getInstanceType();
assertEquals(NATIVE_PROPERTIES_COUNT + 1, classA.getPropertiesCount());
checkObjectType(classA, "m1", NUMBER_TYPE);
}
public void testAddingMethodsPrototypeIdiomAndObjectLiteralSimpleNamespace()
throws Exception {
Node js1Node = parseAndTypeCheck(
"/** @constructor */function A() {}" +
"A.prototype = {m1: 5, m2: true}");
ObjectType instanceType = getInstanceType(js1Node);
assertEquals(NATIVE_PROPERTIES_COUNT + 2,
instanceType.getPropertiesCount());
checkObjectType(instanceType, "m1", NUMBER_TYPE);
checkObjectType(instanceType, "m2", BOOLEAN_TYPE);
}
public void testDontAddMethodsIfNoConstructor()
throws Exception {
Node js1Node = parseAndTypeCheck(
"function A() {}" +
"A.prototype = {m1: 5, m2: true}");
JSType functionAType = js1Node.getFirstChild().getJSType();
assertEquals("function (): undefined", functionAType.toString());
assertTypeEquals(UNKNOWN_TYPE,
U2U_FUNCTION_TYPE.getPropertyType("m1"));
assertTypeEquals(UNKNOWN_TYPE,
U2U_FUNCTION_TYPE.getPropertyType("m2"));
}
public void testFunctionAssignement() throws Exception {
testTypes("/**" +
"* @param {string} ph0" +
"* @param {string} ph1" +
"* @return {string}" +
"*/" +
"function MSG_CALENDAR_ACCESS_ERROR(ph0, ph1) {return ''}" +
"/** @type {Function} */" +
"var MSG_CALENDAR_ADD_ERROR = MSG_CALENDAR_ACCESS_ERROR;");
}
public void testAddMethodsPrototypeTwoWays() throws Exception {
Node js1Node = parseAndTypeCheck(
"/** @constructor */function A() {}" +
"A.prototype = {m1: 5, m2: true};" +
"A.prototype.m3 = 'third property!';");
ObjectType instanceType = getInstanceType(js1Node);
assertEquals("A", instanceType.toString());
assertEquals(NATIVE_PROPERTIES_COUNT + 3,
instanceType.getPropertiesCount());
checkObjectType(instanceType, "m1", NUMBER_TYPE);
checkObjectType(instanceType, "m2", BOOLEAN_TYPE);
checkObjectType(instanceType, "m3", STRING_TYPE);
}
public void testPrototypePropertyTypes() throws Exception {
Node js1Node = parseAndTypeCheck(
"/** @constructor */function A() {\n" +
" /** @type string */ this.m1;\n" +
" /** @type Object? */ this.m2 = {};\n" +
" /** @type boolean */ this.m3;\n" +
"}\n" +
"/** @type string */ A.prototype.m4;\n" +
"/** @type number */ A.prototype.m5 = 0;\n" +
"/** @type boolean */ A.prototype.m6;\n");
ObjectType instanceType = getInstanceType(js1Node);
assertEquals(NATIVE_PROPERTIES_COUNT + 6,
instanceType.getPropertiesCount());
checkObjectType(instanceType, "m1", STRING_TYPE);
checkObjectType(instanceType, "m2",
createUnionType(OBJECT_TYPE, NULL_TYPE));
checkObjectType(instanceType, "m3", BOOLEAN_TYPE);
checkObjectType(instanceType, "m4", STRING_TYPE);
checkObjectType(instanceType, "m5", NUMBER_TYPE);
checkObjectType(instanceType, "m6", BOOLEAN_TYPE);
}
public void testValueTypeBuiltInPrototypePropertyType() throws Exception {
Node node = parseAndTypeCheck("\"x\".charAt(0)");
assertTypeEquals(STRING_TYPE, node.getFirstChild().getFirstChild().getJSType());
}
public void testDeclareBuiltInConstructor() throws Exception {
// Built-in prototype properties should be accessible
// even if the built-in constructor is declared.
Node node = parseAndTypeCheck(
"/** @constructor */ var String = function(opt_str) {};\n" +
"(new String(\"x\")).charAt(0)");
assertTypeEquals(STRING_TYPE, node.getLastChild().getFirstChild().getJSType());
}
public void testExtendBuiltInType1() throws Exception {
String externs =
"/** @constructor */ var String = function(opt_str) {};\n" +
"/**\n" +
"* @param {number} start\n" +
"* @param {number} opt_length\n" +
"* @return {string}\n" +
"*/\n" +
"String.prototype.substr = function(start, opt_length) {};\n";
Node n1 = parseAndTypeCheck(externs + "(new String(\"x\")).substr(0,1);");
assertTypeEquals(STRING_TYPE, n1.getLastChild().getFirstChild().getJSType());
}
public void testExtendBuiltInType2() throws Exception {
String externs =
"/** @constructor */ var String = function(opt_str) {};\n" +
"/**\n" +
"* @param {number} start\n" +
"* @param {number} opt_length\n" +
"* @return {string}\n" +
"*/\n" +
"String.prototype.substr = function(start, opt_length) {};\n";
Node n2 = parseAndTypeCheck(externs + "\"x\".substr(0,1);");
assertTypeEquals(STRING_TYPE, n2.getLastChild().getFirstChild().getJSType());
}
public void testExtendFunction1() throws Exception {
Node n = parseAndTypeCheck("/**@return {number}*/Function.prototype.f = " +
"function() { return 1; };\n" +
"(new Function()).f();");
JSType type = n.getLastChild().getLastChild().getJSType();
assertTypeEquals(NUMBER_TYPE, type);
}
public void testExtendFunction2() throws Exception {
Node n = parseAndTypeCheck("/**@return {number}*/Function.prototype.f = " +
"function() { return 1; };\n" +
"(function() {}).f();");
JSType type = n.getLastChild().getLastChild().getJSType();
assertTypeEquals(NUMBER_TYPE, type);
}
public void testInheritanceCheck1() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"Sub.prototype.foo = function() {};");
}
public void testInheritanceCheck2() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override */Sub.prototype.foo = function() {};",
"property foo not defined on any superclass of Sub");
}
public void testInheritanceCheck3() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"Super.prototype.foo = function() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"Sub.prototype.foo = function() {};",
"property foo already defined on superclass Super; " +
"use @override to override it");
}
public void testInheritanceCheck4() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"Super.prototype.foo = function() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override */Sub.prototype.foo = function() {};");
}
public void testInheritanceCheck5() throws Exception {
testTypes(
"/** @constructor */function Root() {};" +
"Root.prototype.foo = function() {};" +
"/** @constructor\n @extends {Root} */function Super() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"Sub.prototype.foo = function() {};",
"property foo already defined on superclass Root; " +
"use @override to override it");
}
public void testInheritanceCheck6() throws Exception {
testTypes(
"/** @constructor */function Root() {};" +
"Root.prototype.foo = function() {};" +
"/** @constructor\n @extends {Root} */function Super() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override */Sub.prototype.foo = function() {};");
}
public void testInheritanceCheck7() throws Exception {
testTypes(
"var goog = {};" +
"/** @constructor */goog.Super = function() {};" +
"goog.Super.prototype.foo = 3;" +
"/** @constructor\n @extends {goog.Super} */goog.Sub = function() {};" +
"goog.Sub.prototype.foo = 5;");
}
public void testInheritanceCheck8() throws Exception {
testTypes(
"var goog = {};" +
"/** @constructor */goog.Super = function() {};" +
"goog.Super.prototype.foo = 3;" +
"/** @constructor\n @extends {goog.Super} */goog.Sub = function() {};" +
"/** @override */goog.Sub.prototype.foo = 5;");
}
public void testInheritanceCheck9_1() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"Super.prototype.foo = function() { return 3; };" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override\n @return {number} */Sub.prototype.foo =\n" +
"function() { return 1; };");
}
public void testInheritanceCheck9_2() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"/** @return {number} */" +
"Super.prototype.foo = function() { return 1; };" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override */Sub.prototype.foo =\n" +
"function() {};");
}
public void testInheritanceCheck9_3() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"/** @return {number} */" +
"Super.prototype.foo = function() { return 1; };" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override\n @return {string} */Sub.prototype.foo =\n" +
"function() { return \"some string\" };",
"mismatch of the foo property type and the type of the property it " +
"overrides from superclass Super\n" +
"original: function (this:Super): number\n" +
"override: function (this:Sub): string");
}
public void testInheritanceCheck10_1() throws Exception {
testTypes(
"/** @constructor */function Root() {};" +
"Root.prototype.foo = function() { return 3; };" +
"/** @constructor\n @extends {Root} */function Super() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override\n @return {number} */Sub.prototype.foo =\n" +
"function() { return 1; };");
}
public void testInheritanceCheck10_2() throws Exception {
testTypes(
"/** @constructor */function Root() {};" +
"/** @return {number} */" +
"Root.prototype.foo = function() { return 1; };" +
"/** @constructor\n @extends {Root} */function Super() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override */Sub.prototype.foo =\n" +
"function() {};");
}
public void testInheritanceCheck10_3() throws Exception {
testTypes(
"/** @constructor */function Root() {};" +
"/** @return {number} */" +
"Root.prototype.foo = function() { return 1; };" +
"/** @constructor\n @extends {Root} */function Super() {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override\n @return {string} */Sub.prototype.foo =\n" +
"function() { return \"some string\" };",
"mismatch of the foo property type and the type of the property it " +
"overrides from superclass Root\n" +
"original: function (this:Root): number\n" +
"override: function (this:Sub): string");
}
public void testInterfaceInheritanceCheck11() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"/** @param {number} bar */Super.prototype.foo = function(bar) {};" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override\n @param {string} bar */Sub.prototype.foo =\n" +
"function(bar) {};",
"mismatch of the foo property type and the type of the property it " +
"overrides from superclass Super\n" +
"original: function (this:Super, number): undefined\n" +
"override: function (this:Sub, string): undefined");
}
public void testInheritanceCheck12() throws Exception {
testTypes(
"var goog = {};" +
"/** @constructor */goog.Super = function() {};" +
"goog.Super.prototype.foo = 3;" +
"/** @constructor\n @extends {goog.Super} */goog.Sub = function() {};" +
"/** @override */goog.Sub.prototype.foo = \"some string\";");
}
public void testInheritanceCheck13() throws Exception {
testTypes(
"var goog = {};\n" +
"/** @constructor\n @extends {goog.Missing} */function Sub() {};" +
"/** @override */Sub.prototype.foo = function() {};",
"Bad type annotation. Unknown type goog.Missing");
}
public void testInheritanceCheck14() throws Exception {
testClosureTypes(
"var goog = {};\n" +
"/** @constructor\n @extends {goog.Missing} */\n" +
"goog.Super = function() {};\n" +
"/** @constructor\n @extends {goog.Super} */function Sub() {};" +
"/** @override */Sub.prototype.foo = function() {};",
"Bad type annotation. Unknown type goog.Missing");
}
public void testInheritanceCheck15() throws Exception {
testTypes(
"/** @constructor */function Super() {};" +
"/** @param {number} bar */Super.prototype.foo;" +
"/** @constructor\n @extends {Super} */function Sub() {};" +
"/** @override\n @param {number} bar */Sub.prototype.foo =\n" +
"function(bar) {};");
}
public void testInheritanceCheck16() throws Exception {
testTypes(
"var goog = {};" +
"/** @constructor */goog.Super = function() {};" +
"/** @type {number} */ goog.Super.prototype.foo = 3;" +
"/** @constructor\n @extends {goog.Super} */goog.Sub = function() {};" +
"/** @type {number} */ goog.Sub.prototype.foo = 5;",
"property foo already defined on superclass goog.Super; " +
"use @override to override it");
}
public void testInheritanceCheck17() throws Exception {
// Make sure this warning still works, even when there's no
// @override tag.
reportMissingOverrides = CheckLevel.OFF;
testTypes(
"var goog = {};" +
"/** @constructor */goog.Super = function() {};" +
"/** @param {number} x */ goog.Super.prototype.foo = function(x) {};" +
"/** @constructor\n @extends {goog.Super} */goog.Sub = function() {};" +
"/** @param {string} x */ goog.Sub.prototype.foo = function(x) {};",
"mismatch of the foo property type and the type of the property it " +
"overrides from superclass goog.Super\n" +
"original: function (this:goog.Super, number): undefined\n" +
"override: function (this:goog.Sub, string): undefined");
}
public void testInterfacePropertyOverride1() throws Exception {
testTypes(
"/** @interface */function Super() {};" +
"/** @desc description */Super.prototype.foo = function() {};" +
"/** @interface\n @extends {Super} */function Sub() {};" +
"/** @desc description */Sub.prototype.foo = function() {};");
}
public void testInterfacePropertyOverride2() throws Exception {
testTypes(
"/** @interface */function Root() {};" +
"/** @desc description */Root.prototype.foo = function() {};" +
"/** @interface\n @extends {Root} */function Super() {};" +
"/** @interface\n @extends {Super} */function Sub() {};" +
"/** @desc description */Sub.prototype.foo = function() {};");
}
public void testInterfaceInheritanceCheck1() throws Exception {
testTypes(
"/** @interface */function Super() {};" +
"/** @desc description */Super.prototype.foo = function() {};" +
"/** @constructor\n @implements {Super} */function Sub() {};" +
"Sub.prototype.foo = function() {};",
"property foo already defined on interface Super; use @override to " +
"override it");
}
public void testInterfaceInheritanceCheck2() throws Exception {
testTypes(
"/** @interface */function Super() {};" +
"/** @desc description */Super.prototype.foo = function() {};" +
"/** @constructor\n @implements {Super} */function Sub() {};" +
"/** @override */Sub.prototype.foo = function() {};");
}
public void testInterfaceInheritanceCheck3() throws Exception {
testTypes(
"/** @interface */function Root() {};" +
"/** @return {number} */Root.prototype.foo = function() {};" +
"/** @interface\n @extends {Root} */function Super() {};" +
"/** @constructor\n @implements {Super} */function Sub() {};" +
"/** @return {number} */Sub.prototype.foo = function() { return 1;};",
"property foo already defined on interface Root; use @override to " +
"override it");
}
public void testInterfaceInheritanceCheck4() throws Exception {
testTypes(
"/** @interface */function Root() {};" +
"/** @return {number} */Root.prototype.foo = function() {};" +
"/** @interface\n @extends {Root} */function Super() {};" +
"/** @constructor\n @implements {Super} */function Sub() {};" +
"/** @override\n * @return {number} */Sub.prototype.foo =\n" +
"function() { return 1;};");
}
public void testInterfaceInheritanceCheck5() throws Exception {
testTypes(
"/** @interface */function Super() {};" +
"/** @return {string} */Super.prototype.foo = function() {};" +
"/** @constructor\n @implements {Super} */function Sub() {};" +
"/** @override\n @return {number} */Sub.prototype.foo =\n" +
"function() { return 1; };",
"mismatch of the foo property type and the type of the property it " +
"overrides from interface Super\n" +
"original: function (this:Super): string\n" +
"override: function (this:Sub): number");
}
public void testInterfaceInheritanceCheck6() throws Exception {
testTypes(
"/** @interface */function Root() {};" +
"/** @return {string} */Root.prototype.foo = function() {};" +
"/** @interface\n @extends {Root} */function Super() {};" +
"/** @constructor\n @implements {Super} */function Sub() {};" +
"/** @override\n @return {number} */Sub.prototype.foo =\n" +
"function() { return 1; };",
"mismatch of the foo property type and the type of the property it " +
"overrides from interface Root\n" +
"original: function (this:Root): string\n" +
"override: function (this:Sub): number");
}
public void testInterfaceInheritanceCheck7() throws Exception {
testTypes(
"/** @interface */function Super() {};" +
"/** @param {number} bar */Super.prototype.foo = function(bar) {};" +
"/** @constructor\n @implements {Super} */function Sub() {};" +
"/** @override\n @param {string} bar */Sub.prototype.foo =\n" +
"function(bar) {};",
"mismatch of the foo property type and the type of the property it " +
"overrides from interface Super\n" +
"original: function (this:Super, number): undefined\n" +
"override: function (this:Sub, string): undefined");
}
public void testInterfaceInheritanceCheck8() throws Exception {
testTypes(
"/** @constructor\n @implements {Super} */function Sub() {};" +
"/** @override */Sub.prototype.foo = function() {};",
new String[] {
"Bad type annotation. Unknown type Super",
"property foo not defined on any superclass of Sub"
});
}
public void testInterfaceInheritanceCheck9() throws Exception {
testTypes(
"/** @interface */ function I() {}" +
"/** @return {number} */ I.prototype.bar = function() {};" +
"/** @constructor */ function F() {}" +
"/** @return {number} */ F.prototype.bar = function() {return 3; };" +
"/** @return {number} */ F.prototype.foo = function() {return 3; };" +
"/** @constructor \n * @extends {F} \n * @implements {I} */ " +
"function G() {}" +
"/** @return {string} */ function f() { return new G().bar(); }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testInterfaceInheritanceCheck10() throws Exception {
testTypes(
"/** @interface */ function I() {}" +
"/** @return {number} */ I.prototype.bar = function() {};" +
"/** @constructor */ function F() {}" +
"/** @return {number} */ F.prototype.foo = function() {return 3; };" +
"/** @constructor \n * @extends {F} \n * @implements {I} */ " +
"function G() {}" +
"/** @return {number} \n * @override */ " +
"G.prototype.bar = G.prototype.foo;" +
"/** @return {string} */ function f() { return new G().bar(); }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testInterfaceInheritanceCheck12() throws Exception {
testTypes(
"/** @interface */ function I() {};\n" +
"/** @type {string} */ I.prototype.foobar;\n" +
"/** \n * @constructor \n * @implements {I} */\n" +
"function C() {\n" +
"/** \n * @type {number} */ this.foobar = 2;};\n" +
"/** @type {I} */ \n var test = new C(); alert(test.foobar);",
"mismatch of the foobar property type and the type of the property" +
" it overrides from interface I\n" +
"original: string\n" +
"override: number");
}
public void testInterfaceInheritanceCheck13() throws Exception {
testTypes(
"function abstractMethod() {};\n" +
"/** @interface */var base = function() {};\n" +
"/** @extends {base} \n @interface */ var Int = function() {}\n" +
"/** @type {{bar : !Function}} */ var x; \n" +
"/** @type {!Function} */ base.prototype.bar = abstractMethod; \n" +
"/** @type {Int} */ var foo;\n" +
"foo.bar();");
}
public void testInterfacePropertyNotImplemented() throws Exception {
testTypes(
"/** @interface */function Int() {};" +
"/** @desc description */Int.prototype.foo = function() {};" +
"/** @constructor\n @implements {Int} */function Foo() {};",
"property foo on interface Int is not implemented by type Foo");
}
public void testInterfacePropertyNotImplemented2() throws Exception {
testTypes(
"/** @interface */function Int() {};" +
"/** @desc description */Int.prototype.foo = function() {};" +
"/** @interface \n @extends {Int} */function Int2() {};" +
"/** @constructor\n @implements {Int2} */function Foo() {};",
"property foo on interface Int is not implemented by type Foo");
}
public void testStubConstructorImplementingInterface() throws Exception {
// This does not throw a warning for unimplemented property because Foo is
// just a stub.
testTypes(
// externs
"/** @interface */ function Int() {}\n" +
"/** @desc description */Int.prototype.foo = function() {};" +
"/** @constructor \n @implements {Int} */ var Foo;\n",
"", null, false);
}
public void testObjectLiteral() throws Exception {
Node n = parseAndTypeCheck("var a = {m1: 7, m2: 'hello'}");
Node nameNode = n.getFirstChild().getFirstChild();
Node objectNode = nameNode.getFirstChild();
// node extraction
assertEquals(Token.NAME, nameNode.getType());
assertEquals(Token.OBJECTLIT, objectNode.getType());
// value's type
ObjectType objectType =
(ObjectType) objectNode.getJSType();
assertTypeEquals(NUMBER_TYPE, objectType.getPropertyType("m1"));
assertTypeEquals(STRING_TYPE, objectType.getPropertyType("m2"));
// variable's type
assertTypeEquals(objectType, nameNode.getJSType());
}
public void testObjectLiteralDeclaration1() throws Exception {
testTypes(
"var x = {" +
"/** @type {boolean} */ abc: true," +
"/** @type {number} */ 'def': 0," +
"/** @type {string} */ 3: 'fgh'" +
"};");
}
public void testObjectLiteralDeclaration2() throws Exception {
testTypes(
"var x = {" +
" /** @type {boolean} */ abc: true" +
"};" +
"x.abc = 0;",
"assignment to property abc of x\n" +
"found : number\n" +
"required: boolean");
}
public void testObjectLiteralDeclaration3() throws Exception {
testTypes(
"/** @param {{foo: !Function}} x */ function f(x) {}" +
"f({foo: function() {}});");
}
public void testObjectLiteralDeclaration4() throws Exception {
testClosureTypes(
"var x = {" +
" /** @param {boolean} x */ abc: function(x) {}" +
"};" +
"/**\n" +
" * @param {string} x\n" +
" * @suppress {duplicate}\n" +
" */ x.abc = function(x) {};",
"assignment to property abc of x\n" +
"found : function (string): undefined\n" +
"required: function (boolean): undefined");
// TODO(user): suppress {duplicate} currently also silence the
// redefining type error in the TypeValidator. Maybe it needs
// a new suppress name instead?
}
public void testObjectLiteralDeclaration5() throws Exception {
testTypes(
"var x = {" +
" /** @param {boolean} x */ abc: function(x) {}" +
"};" +
"/**\n" +
" * @param {boolean} x\n" +
" * @suppress {duplicate}\n" +
" */ x.abc = function(x) {};");
}
public void testObjectLiteralDeclaration6() throws Exception {
testTypes(
"var x = {};" +
"/**\n" +
" * @param {boolean} x\n" +
" * @suppress {duplicate}\n" +
" */ x.abc = function(x) {};" +
"x = {" +
" /**\n" +
" * @param {boolean} x\n" +
" * @suppress {duplicate}\n" +
" */" +
" abc: function(x) {}" +
"};");
}
public void testObjectLiteralDeclaration7() throws Exception {
testTypes(
"var x = {};" +
"/**\n" +
" * @type {function(boolean): undefined}\n" +
" */ x.abc = function(x) {};" +
"x = {" +
" /**\n" +
" * @param {boolean} x\n" +
" * @suppress {duplicate}\n" +
" */" +
" abc: function(x) {}" +
"};");
}
public void testCallDateConstructorAsFunction() throws Exception {
// ECMA-262 15.9.2: When Date is called as a function rather than as a
// constructor, it returns a string.
Node n = parseAndTypeCheck("Date()");
assertTypeEquals(STRING_TYPE, n.getFirstChild().getFirstChild().getJSType());
}
// According to ECMA-262, Error & Array function calls are equivalent to
// constructor calls.
public void testCallErrorConstructorAsFunction() throws Exception {
Node n = parseAndTypeCheck("Error('x')");
assertTypeEquals(ERROR_TYPE,
n.getFirstChild().getFirstChild().getJSType());
}
public void testCallArrayConstructorAsFunction() throws Exception {
Node n = parseAndTypeCheck("Array()");
assertTypeEquals(ARRAY_TYPE,
n.getFirstChild().getFirstChild().getJSType());
}
public void testPropertyTypeOfUnionType() throws Exception {
testTypes("var a = {};" +
"/** @constructor */ a.N = function() {};\n" +
"a.N.prototype.p = 1;\n" +
"/** @constructor */ a.S = function() {};\n" +
"a.S.prototype.p = 'a';\n" +
"/** @param {!a.N|!a.S} x\n@return {string} */\n" +
"var f = function(x) { return x.p; };",
"inconsistent return type\n" +
"found : (number|string)\n" +
"required: string");
}
// TODO(user): We should flag these as invalid. This will probably happen
// when we make sure the interface is never referenced outside of its
// definition. We might want more specific and helpful error messages.
//public void testWarningOnInterfacePrototype() throws Exception {
// testTypes("/** @interface */ u.T = function() {};\n" +
// "/** @return {number} */ u.T.prototype = function() { };",
// "e of its definition");
//}
//
//public void testBadPropertyOnInterface1() throws Exception {
// testTypes("/** @interface */ u.T = function() {};\n" +
// "/** @return {number} */ u.T.f = function() { return 1;};",
// "cannot reference an interface outside of its definition");
//}
//
//public void testBadPropertyOnInterface2() throws Exception {
// testTypes("/** @interface */ function T() {};\n" +
// "/** @return {number} */ T.f = function() { return 1;};",
// "cannot reference an interface outside of its definition");
//}
//
//public void testBadPropertyOnInterface3() throws Exception {
// testTypes("/** @interface */ u.T = function() {}; u.T.x",
// "cannot reference an interface outside of its definition");
//}
//
//public void testBadPropertyOnInterface4() throws Exception {
// testTypes("/** @interface */ function T() {}; T.x;",
// "cannot reference an interface outside of its definition");
//}
public void testAnnotatedPropertyOnInterface1() throws Exception {
// For interfaces we must allow function definitions that don't have a
// return statement, even though they declare a returned type.
testTypes("/** @interface */ u.T = function() {};\n" +
"/** @return {number} */ u.T.prototype.f = function() {};");
}
public void testAnnotatedPropertyOnInterface2() throws Exception {
testTypes("/** @interface */ u.T = function() {};\n" +
"/** @return {number} */ u.T.prototype.f = function() { };");
}
public void testAnnotatedPropertyOnInterface3() throws Exception {
testTypes("/** @interface */ function T() {};\n" +
"/** @return {number} */ T.prototype.f = function() { };");
}
public void testAnnotatedPropertyOnInterface4() throws Exception {
testTypes(
CLOSURE_DEFS +
"/** @interface */ function T() {};\n" +
"/** @return {number} */ T.prototype.f = goog.abstractMethod;");
}
// TODO(user): If we want to support this syntax we have to warn about
// missing annotations.
//public void testWarnUnannotatedPropertyOnInterface1() throws Exception {
// testTypes("/** @interface */ u.T = function () {}; u.T.prototype.x;",
// "interface property x is not annotated");
//}
//
//public void testWarnUnannotatedPropertyOnInterface2() throws Exception {
// testTypes("/** @interface */ function T() {}; T.prototype.x;",
// "interface property x is not annotated");
//}
public void testWarnUnannotatedPropertyOnInterface5() throws Exception {
testTypes("/** @interface */ u.T = function () {};\n" +
"/** @desc x does something */u.T.prototype.x = function() {};");
}
public void testWarnUnannotatedPropertyOnInterface6() throws Exception {
testTypes("/** @interface */ function T() {};\n" +
"/** @desc x does something */T.prototype.x = function() {};");
}
// TODO(user): If we want to support this syntax we have to warn about
// the invalid type of the interface member.
//public void testWarnDataPropertyOnInterface1() throws Exception {
// testTypes("/** @interface */ u.T = function () {};\n" +
// "/** @type {number} */u.T.prototype.x;",
// "interface members can only be plain functions");
//}
public void testDataPropertyOnInterface1() throws Exception {
testTypes("/** @interface */ function T() {};\n" +
"/** @type {number} */T.prototype.x;");
}
public void testDataPropertyOnInterface2() throws Exception {
reportMissingOverrides = CheckLevel.OFF;
testTypes("/** @interface */ function T() {};\n" +
"/** @type {number} */T.prototype.x;\n" +
"/** @constructor \n" +
" * @implements {T} \n" +
" */\n" +
"function C() {}\n" +
"C.prototype.x = 'foo';",
"mismatch of the x property type and the type of the property it " +
"overrides from interface T\n" +
"original: number\n" +
"override: string");
}
public void testDataPropertyOnInterface3() throws Exception {
testTypes("/** @interface */ function T() {};\n" +
"/** @type {number} */T.prototype.x;\n" +
"/** @constructor \n" +
" * @implements {T} \n" +
" */\n" +
"function C() {}\n" +
"/** @override */\n" +
"C.prototype.x = 'foo';",
"mismatch of the x property type and the type of the property it " +
"overrides from interface T\n" +
"original: number\n" +
"override: string");
}
public void testDataPropertyOnInterface4() throws Exception {
testTypes("/** @interface */ function T() {};\n" +
"/** @type {number} */T.prototype.x;\n" +
"/** @constructor \n" +
" * @implements {T} \n" +
" */\n" +
"function C() { /** @type {string} */ \n this.x = 'foo'; }\n",
"mismatch of the x property type and the type of the property it " +
"overrides from interface T\n" +
"original: number\n" +
"override: string");
}
public void testWarnDataPropertyOnInterface3() throws Exception {
testTypes("/** @interface */ u.T = function () {};\n" +
"/** @type {number} */u.T.prototype.x = 1;",
"interface members can only be empty property declarations, "
+ "empty functions, or goog.abstractMethod");
}
public void testWarnDataPropertyOnInterface4() throws Exception {
testTypes("/** @interface */ function T() {};\n" +
"/** @type {number} */T.prototype.x = 1;",
"interface members can only be empty property declarations, "
+ "empty functions, or goog.abstractMethod");
}
// TODO(user): If we want to support this syntax we should warn about the
// mismatching types in the two tests below.
//public void testErrorMismatchingPropertyOnInterface1() throws Exception {
// testTypes("/** @interface */ u.T = function () {};\n" +
// "/** @param {Number} foo */u.T.prototype.x =\n" +
// "/** @param {String} foo */function(foo) {};",
// "found : \n" +
// "required: ");
//}
//
//public void testErrorMismatchingPropertyOnInterface2() throws Exception {
// testTypes("/** @interface */ function T() {};\n" +
// "/** @return {number} */T.prototype.x =\n" +
// "/** @return {string} */function() {};",
// "found : \n" +
// "required: ");
//}
// TODO(user): We should warn about this (bar is missing an annotation). We
// probably don't want to warn about all missing parameter annotations, but
// we should be as strict as possible regarding interfaces.
//public void testErrorMismatchingPropertyOnInterface3() throws Exception {
// testTypes("/** @interface */ u.T = function () {};\n" +
// "/** @param {Number} foo */u.T.prototype.x =\n" +
// "function(foo, bar) {};",
// "found : \n" +
// "required: ");
//}
public void testErrorMismatchingPropertyOnInterface4() throws Exception {
testTypes("/** @interface */ u.T = function () {};\n" +
"/** @param {Number} foo */u.T.prototype.x =\n" +
"function() {};",
"parameter foo does not appear in u.T.prototype.x's parameter list");
}
public void testErrorMismatchingPropertyOnInterface5() throws Exception {
testTypes("/** @interface */ function T() {};\n" +
"/** @type {number} */T.prototype.x = function() { };",
"assignment to property x of T.prototype\n" +
"found : function (): undefined\n" +
"required: number");
}
public void testErrorMismatchingPropertyOnInterface6() throws Exception {
testClosureTypesMultipleWarnings(
"/** @interface */ function T() {};\n" +
"/** @return {number} */T.prototype.x = 1",
Lists.newArrayList(
"assignment to property x of T.prototype\n" +
"found : number\n" +
"required: function (this:T): number",
"interface members can only be empty property declarations, " +
"empty functions, or goog.abstractMethod"));
}
public void testInterfaceNonEmptyFunction() throws Exception {
testTypes("/** @interface */ function T() {};\n" +
"T.prototype.x = function() { return 'foo'; }",
"interface member functions must have an empty body"
);
}
public void testDoubleNestedInterface() throws Exception {
testTypes("/** @interface */ var I1 = function() {};\n" +
"/** @interface */ I1.I2 = function() {};\n" +
"/** @interface */ I1.I2.I3 = function() {};\n");
}
public void testStaticDataPropertyOnNestedInterface() throws Exception {
testTypes("/** @interface */ var I1 = function() {};\n" +
"/** @interface */ I1.I2 = function() {};\n" +
"/** @type {number} */ I1.I2.x = 1;\n");
}
public void testInterfaceInstantiation() throws Exception {
testTypes("/** @interface */var f = function(){}; new f",
"cannot instantiate non-constructor");
}
public void testPrototypeLoop() throws Exception {
testClosureTypesMultipleWarnings(
suppressMissingProperty("foo") +
"/** @constructor \n * @extends {T} */var T = function() {};" +
"alert((new T).foo);",
Lists.newArrayList(
"Parse error. Cycle detected in inheritance chain of type T",
"Could not resolve type in @extends tag of T"));
}
public void testImplementsLoop() throws Exception {
testClosureTypesMultipleWarnings(
suppressMissingProperty("foo") +
"/** @constructor \n * @implements {T} */var T = function() {};" +
"alert((new T).foo);",
Lists.newArrayList(
"Parse error. Cycle detected in inheritance chain of type T"));
}
public void testImplementsExtendsLoop() throws Exception {
testClosureTypesMultipleWarnings(
suppressMissingProperty("foo") +
"/** @constructor \n * @implements {F} */var G = function() {};" +
"/** @constructor \n * @extends {G} */var F = function() {};" +
"alert((new F).foo);",
Lists.newArrayList(
"Parse error. Cycle detected in inheritance chain of type F"));
}
public void testInterfaceExtendsLoop() throws Exception {
// TODO(user): This should give a cycle in inheritance graph error,
// not a cannot resolve error.
testClosureTypesMultipleWarnings(
suppressMissingProperty("foo") +
"/** @interface \n * @extends {F} */var G = function() {};" +
"/** @interface \n * @extends {G} */var F = function() {};",
Lists.newArrayList(
"Could not resolve type in @extends tag of G"));
}
public void testConversionFromInterfaceToRecursiveConstructor()
throws Exception {
testClosureTypesMultipleWarnings(
suppressMissingProperty("foo") +
"/** @interface */ var OtherType = function() {}\n" +
"/** @implements {MyType} \n * @constructor */\n" +
"var MyType = function() {}\n" +
"/** @type {MyType} */\n" +
"var x = /** @type {!OtherType} */ (new Object());",
Lists.newArrayList(
"Parse error. Cycle detected in inheritance chain of type MyType",
"initializing variable\n" +
"found : OtherType\n" +
"required: (MyType|null)"));
}
public void testDirectPrototypeAssign() throws Exception {
// For now, we just ignore @type annotations on the prototype.
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @constructor */ function Bar() {}" +
"/** @type {Array} */ Bar.prototype = new Foo()");
}
// In all testResolutionViaRegistry* tests, since u is unknown, u.T can only
// be resolved via the registry and not via properties.
public void testResolutionViaRegistry1() throws Exception {
testTypes("/** @constructor */ u.T = function() {};\n" +
"/** @type {(number|string)} */ u.T.prototype.a;\n" +
"/**\n" +
"* @param {u.T} t\n" +
"* @return {string}\n" +
"*/\n" +
"var f = function(t) { return t.a; };",
"inconsistent return type\n" +
"found : (number|string)\n" +
"required: string");
}
public void testResolutionViaRegistry2() throws Exception {
testTypes(
"/** @constructor */ u.T = function() {" +
" this.a = 0; };\n" +
"/**\n" +
"* @param {u.T} t\n" +
"* @return {string}\n" +
"*/\n" +
"var f = function(t) { return t.a; };",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testResolutionViaRegistry3() throws Exception {
testTypes("/** @constructor */ u.T = function() {};\n" +
"/** @type {(number|string)} */ u.T.prototype.a = 0;\n" +
"/**\n" +
"* @param {u.T} t\n" +
"* @return {string}\n" +
"*/\n" +
"var f = function(t) { return t.a; };",
"inconsistent return type\n" +
"found : (number|string)\n" +
"required: string");
}
public void testResolutionViaRegistry4() throws Exception {
testTypes("/** @constructor */ u.A = function() {};\n" +
"/**\n* @constructor\n* @extends {u.A}\n*/\nu.A.A = function() {}\n;" +
"/**\n* @constructor\n* @extends {u.A}\n*/\nu.A.B = function() {};\n" +
"var ab = new u.A.B();\n" +
"/** @type {!u.A} */ var a = ab;\n" +
"/** @type {!u.A.A} */ var aa = ab;\n",
"initializing variable\n" +
"found : u.A.B\n" +
"required: u.A.A");
}
public void testResolutionViaRegistry5() throws Exception {
Node n = parseAndTypeCheck("/** @constructor */ u.T = function() {}; u.T");
JSType type = n.getLastChild().getLastChild().getJSType();
assertFalse(type.isUnknownType());
assertTrue(type instanceof FunctionType);
assertEquals("u.T",
((FunctionType) type).getInstanceType().getReferenceName());
}
public void testGatherProperyWithoutAnnotation1() throws Exception {
Node n = parseAndTypeCheck("/** @constructor */ var T = function() {};" +
"/** @type {!T} */var t; t.x; t;");
JSType type = n.getLastChild().getLastChild().getJSType();
assertFalse(type.isUnknownType());
assertTrue(type instanceof ObjectType);
ObjectType objectType = (ObjectType) type;
assertFalse(objectType.hasProperty("x"));
Asserts.assertTypeCollectionEquals(
Lists.newArrayList(objectType),
registry.getTypesWithProperty("x"));
}
public void testGatherProperyWithoutAnnotation2() throws Exception {
TypeCheckResult ns =
parseAndTypeCheckWithScope("/** @type {!Object} */var t; t.x; t;");
Node n = ns.root;
Scope s = ns.scope;
JSType type = n.getLastChild().getLastChild().getJSType();
assertFalse(type.isUnknownType());
assertTypeEquals(type, OBJECT_TYPE);
assertTrue(type instanceof ObjectType);
ObjectType objectType = (ObjectType) type;
assertFalse(objectType.hasProperty("x"));
Asserts.assertTypeCollectionEquals(
Lists.newArrayList(OBJECT_TYPE),
registry.getTypesWithProperty("x"));
}
public void testFunctionMasksVariableBug() throws Exception {
testTypes("var x = 4; var f = function x(b) { return b ? 1 : x(true); };",
"function x masks variable (IE bug)");
}
public void testDfa1() throws Exception {
testTypes("var x = null;\n x = 1;\n /** @type number */ var y = x;");
}
public void testDfa2() throws Exception {
testTypes("function u() {}\n" +
"/** @return {number} */ function f() {\nvar x = 'todo';\n" +
"if (u()) { x = 1; } else { x = 2; } return x;\n}");
}
public void testDfa3() throws Exception {
testTypes("function u() {}\n" +
"/** @return {number} */ function f() {\n" +
"/** @type {number|string} */ var x = 'todo';\n" +
"if (u()) { x = 1; } else { x = 2; } return x;\n}");
}
public void testDfa4() throws Exception {
testTypes("/** @param {Date?} d */ function f(d) {\n" +
"if (!d) { return; }\n" +
"/** @type {!Date} */ var e = d;\n}");
}
public void testDfa5() throws Exception {
testTypes("/** @return {string?} */ function u() {return 'a';}\n" +
"/** @param {string?} x\n@return {string} */ function f(x) {\n" +
"while (!x) { x = u(); }\nreturn x;\n}");
}
public void testDfa6() throws Exception {
testTypes("/** @return {Object?} */ function u() {return {};}\n" +
"/** @param {Object?} x */ function f(x) {\n" +
"while (x) { x = u(); if (!x) { x = u(); } }\n}");
}
public void testDfa7() throws Exception {
testTypes("/** @constructor */ var T = function() {};\n" +
"/** @type {Date?} */ T.prototype.x = null;\n" +
"/** @param {!T} t */ function f(t) {\n" +
"if (!t.x) { return; }\n" +
"/** @type {!Date} */ var e = t.x;\n}");
}
public void testDfa8() throws Exception {
testTypes("/** @constructor */ var T = function() {};\n" +
"/** @type {number|string} */ T.prototype.x = '';\n" +
"function u() {}\n" +
"/** @param {!T} t\n@return {number} */ function f(t) {\n" +
"if (u()) { t.x = 1; } else { t.x = 2; } return t.x;\n}");
}
public void testDfa9() throws Exception {
testTypes("function f() {\n/** @type {string?} */var x;\nx = null;\n" +
"if (x == null) { return 0; } else { return 1; } }",
"condition always evaluates to true\n" +
"left : null\n" +
"right: null");
}
public void testDfa10() throws Exception {
testTypes("/** @param {null} x */ function g(x) {}" +
"/** @param {string?} x */function f(x) {\n" +
"if (!x) { x = ''; }\n" +
"if (g(x)) { return 0; } else { return 1; } }",
"actual parameter 1 of g does not match formal parameter\n" +
"found : string\n" +
"required: null");
}
public void testDfa11() throws Exception {
testTypes("/** @param {string} opt_x\n@return {string} */\n" +
"function f(opt_x) { if (!opt_x) { " +
"throw new Error('x cannot be empty'); } return opt_x; }");
}
public void testDfa12() throws Exception {
testTypes("/** @param {string} x \n * @constructor \n */" +
"var Bar = function(x) {};" +
"/** @param {string} x */ function g(x) { return true; }" +
"/** @param {string|number} opt_x */ " +
"function f(opt_x) { " +
" if (opt_x) { new Bar(g(opt_x) && 'x'); }" +
"}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : (number|string)\n" +
"required: string");
}
public void testDfa13() throws Exception {
testTypes(
"/**\n" +
" * @param {string} x \n" +
" * @param {number} y \n" +
" * @param {number} z \n" +
" */" +
"function g(x, y, z) {}" +
"function f() { " +
" var x = 'a'; g(x, x = 3, x);" +
"}");
}
public void testTypeInferenceWithCast1() throws Exception {
testTypes(
"/**@return {(number,null,undefined)}*/function u(x) {return null;}" +
"/**@param {number?} x\n@return {number?}*/function f(x) {return x;}" +
"/**@return {number?}*/function g(x) {" +
"var y = /**@type {number?}*/(u(x)); return f(y);}");
}
public void testTypeInferenceWithCast2() throws Exception {
testTypes(
"/**@return {(number,null,undefined)}*/function u(x) {return null;}" +
"/**@param {number?} x\n@return {number?}*/function f(x) {return x;}" +
"/**@return {number?}*/function g(x) {" +
"var y; y = /**@type {number?}*/(u(x)); return f(y);}");
}
public void testTypeInferenceWithCast3() throws Exception {
testTypes(
"/**@return {(number,null,undefined)}*/function u(x) {return 1;}" +
"/**@return {number}*/function g(x) {" +
"return /**@type {number}*/(u(x));}");
}
public void testTypeInferenceWithCast4() throws Exception {
testTypes(
"/**@return {(number,null,undefined)}*/function u(x) {return 1;}" +
"/**@return {number}*/function g(x) {" +
"return /**@type {number}*/(u(x)) && 1;}");
}
public void testTypeInferenceWithCast5() throws Exception {
testTypes(
"/** @param {number} x */ function foo(x) {}" +
"/** @param {{length:*}} y */ function bar(y) {" +
" /** @type {string} */ y.length;" +
" foo(y.length);" +
"}",
"actual parameter 1 of foo does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testTypeInferenceWithClosure1() throws Exception {
testTypes(
"/** @return {boolean} */" +
"function f() {" +
" /** @type {?string} */ var x = null;" +
" function g() { x = 'y'; } g(); " +
" return x == null;" +
"}");
}
public void testTypeInferenceWithClosure2() throws Exception {
testTypes(
"/** @return {boolean} */" +
"function f() {" +
" /** @type {?string} */ var x = null;" +
" function g() { x = 'y'; } g(); " +
" return x === 3;" +
"}",
"condition always evaluates to false\n" +
"left : (null|string)\n" +
"right: number");
}
public void testTypeInferenceWithNoEntry1() throws Exception {
testTypes(
"/** @param {number} x */ function f(x) {}" +
"/** @constructor */ function Foo() {}" +
"Foo.prototype.init = function() {" +
" /** @type {?{baz: number}} */ this.bar = {baz: 3};" +
"};" +
"/**\n" +
" * @extends {Foo}\n" +
" * @constructor\n" +
" */" +
"function SubFoo() {}" +
"/** Method */" +
"SubFoo.prototype.method = function() {" +
" for (var i = 0; i < 10; i++) {" +
" f(this.bar);" +
" f(this.bar.baz);" +
" }" +
"};",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (null|{baz: number})\n" +
"required: number");
}
public void testTypeInferenceWithNoEntry2() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @param {number} x */ function f(x) {}" +
"/** @param {!Object} x */ function g(x) {}" +
"/** @constructor */ function Foo() {}" +
"Foo.prototype.init = function() {" +
" /** @type {?{baz: number}} */ this.bar = {baz: 3};" +
"};" +
"/**\n" +
" * @extends {Foo}\n" +
" * @constructor\n" +
" */" +
"function SubFoo() {}" +
"/** Method */" +
"SubFoo.prototype.method = function() {" +
" for (var i = 0; i < 10; i++) {" +
" f(this.bar);" +
" goog.asserts.assert(this.bar);" +
" g(this.bar);" +
" }" +
"};",
"actual parameter 1 of f does not match formal parameter\n" +
"found : (null|{baz: number})\n" +
"required: number");
}
public void testForwardPropertyReference() throws Exception {
testTypes("/** @constructor */ var Foo = function() { this.init(); };" +
"/** @return {string} */" +
"Foo.prototype.getString = function() {" +
" return this.number_;" +
"};" +
"Foo.prototype.init = function() {" +
" /** @type {number} */" +
" this.number_ = 3;" +
"};",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testNoForwardTypeDeclaration() throws Exception {
testTypes(
"/** @param {MyType} x */ function f(x) {}",
"Bad type annotation. Unknown type MyType");
}
public void testNoForwardTypeDeclarationAndNoBraces() throws Exception {
testTypes("/** @return The result. */ function f() {}");
}
public void testForwardTypeDeclaration1() throws Exception {
testClosureTypes(
// malformed addDependency calls shouldn't cause a crash
"goog.addDependency();" +
"goog.addDependency('y', [goog]);" +
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/** @param {MyType} x \n * @return {number} */" +
"function f(x) { return 3; }", null);
}
public void testForwardTypeDeclaration2() throws Exception {
String f = "goog.addDependency('zzz.js', ['MyType'], []);" +
"/** @param {MyType} x */ function f(x) { }";
testClosureTypes(f, null);
testClosureTypes(f + "f(3);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: (MyType|null)");
}
public void testForwardTypeDeclaration3() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/** @param {MyType} x */ function f(x) { return x; }" +
"/** @constructor */ var MyType = function() {};" +
"f(3);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: (MyType|null)");
}
public void testForwardTypeDeclaration4() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/** @param {MyType} x */ function f(x) { return x; }" +
"/** @constructor */ var MyType = function() {};" +
"f(new MyType());",
null);
}
public void testForwardTypeDeclaration5() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/**\n" +
" * @constructor\n" +
" * @extends {MyType}\n" +
" */ var YourType = function() {};" +
"/** @override */ YourType.prototype.method = function() {};",
"Could not resolve type in @extends tag of YourType");
}
public void testForwardTypeDeclaration6() throws Exception {
testClosureTypesMultipleWarnings(
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/**\n" +
" * @constructor\n" +
" * @implements {MyType}\n" +
" */ var YourType = function() {};" +
"/** @override */ YourType.prototype.method = function() {};",
Lists.newArrayList(
"Could not resolve type in @implements tag of YourType",
"property method not defined on any superclass of YourType"));
}
public void testForwardTypeDeclaration7() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/** @param {MyType=} x */" +
"function f(x) { return x == undefined; }", null);
}
public void testForwardTypeDeclaration8() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/** @param {MyType} x */" +
"function f(x) { return x.name == undefined; }", null);
}
public void testForwardTypeDeclaration9() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/** @param {MyType} x */" +
"function f(x) { x.name = 'Bob'; }", null);
}
public void testForwardTypeDeclaration10() throws Exception {
String f = "goog.addDependency('zzz.js', ['MyType'], []);" +
"/** @param {MyType|number} x */ function f(x) { }";
testClosureTypes(f, null);
testClosureTypes(f + "f(3);", null);
testClosureTypes(f + "f('3');",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: (MyType|null|number)");
}
public void testForwardTypeDeclaration12() throws Exception {
// We assume that {Function} types can produce anything, and don't
// want to type-check them.
testClosureTypes(
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/**\n" +
" * @param {!Function} ctor\n" +
" * @return {MyType}\n" +
" */\n" +
"function f(ctor) { return new ctor(); }", null);
}
public void testForwardTypeDeclaration13() throws Exception {
// Some projects use {Function} registries to register constructors
// that aren't in their binaries. We want to make sure we can pass these
// around, but still do other checks on them.
testClosureTypes(
"goog.addDependency('zzz.js', ['MyType'], []);" +
"/**\n" +
" * @param {!Function} ctor\n" +
" * @return {MyType}\n" +
" */\n" +
"function f(ctor) { return (new ctor()).impossibleProp; }",
"Property impossibleProp never defined on ?");
}
public void testDuplicateTypeDef() throws Exception {
testTypes(
"var goog = {};" +
"/** @constructor */ goog.Bar = function() {};" +
"/** @typedef {number} */ goog.Bar;",
"variable goog.Bar redefined with type None, " +
"original definition at [testcode]:1 " +
"with type function (new:goog.Bar): undefined");
}
public void testTypeDef1() throws Exception {
testTypes(
"var goog = {};" +
"/** @typedef {number} */ goog.Bar;" +
"/** @param {goog.Bar} x */ function f(x) {}" +
"f(3);");
}
public void testTypeDef2() throws Exception {
testTypes(
"var goog = {};" +
"/** @typedef {number} */ goog.Bar;" +
"/** @param {goog.Bar} x */ function f(x) {}" +
"f('3');",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testTypeDef3() throws Exception {
testTypes(
"var goog = {};" +
"/** @typedef {number} */ var Bar;" +
"/** @param {Bar} x */ function f(x) {}" +
"f('3');",
"actual parameter 1 of f does not match formal parameter\n" +
"found : string\n" +
"required: number");
}
public void testTypeDef4() throws Exception {
testTypes(
"/** @constructor */ function A() {}" +
"/** @constructor */ function B() {}" +
"/** @typedef {(A|B)} */ var AB;" +
"/** @param {AB} x */ function f(x) {}" +
"f(new A()); f(new B()); f(1);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: (A|B|null)");
}
public void testTypeDef5() throws Exception {
// Notice that the error message is slightly different than
// the one for testTypeDef4, even though they should be the same.
// This is an implementation detail necessary for NamedTypes work out
// OK, and it should change if NamedTypes ever go away.
testTypes(
"/** @param {AB} x */ function f(x) {}" +
"/** @constructor */ function A() {}" +
"/** @constructor */ function B() {}" +
"/** @typedef {(A|B)} */ var AB;" +
"f(new A()); f(new B()); f(1);",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: (A|B|null)");
}
public void testCircularTypeDef() throws Exception {
testTypes(
"var goog = {};" +
"/** @typedef {number|Array.<goog.Bar>} */ goog.Bar;" +
"/** @param {goog.Bar} x */ function f(x) {}" +
"f(3); f([3]); f([[3]]);");
}
public void testGetTypedPercent1() throws Exception {
String js = "var id = function(x) { return x; }\n" +
"var id2 = function(x) { return id(x); }";
assertEquals(50.0, getTypedPercent(js), 0.1);
}
public void testGetTypedPercent2() throws Exception {
String js = "var x = {}; x.y = 1;";
assertEquals(100.0, getTypedPercent(js), 0.1);
}
public void testGetTypedPercent3() throws Exception {
String js = "var f = function(x) { x.a = x.b; }";
assertEquals(50.0, getTypedPercent(js), 0.1);
}
public void testGetTypedPercent4() throws Exception {
String js = "var n = {};\n /** @constructor */ n.T = function() {};\n" +
"/** @type n.T */ var x = new n.T();";
assertEquals(100.0, getTypedPercent(js), 0.1);
}
public void testGetTypedPercent5() throws Exception {
String js = "/** @enum {number} */ keys = {A: 1,B: 2,C: 3};";
assertEquals(100.0, getTypedPercent(js), 0.1);
}
public void testGetTypedPercent6() throws Exception {
String js = "a = {TRUE: 1, FALSE: 0};";
assertEquals(100.0, getTypedPercent(js), 0.1);
}
private double getTypedPercent(String js) throws Exception {
Node n = compiler.parseTestCode(js);
Node externs = new Node(Token.BLOCK);
Node externAndJsRoot = new Node(Token.BLOCK, externs, n);
externAndJsRoot.setIsSyntheticBlock(true);
TypeCheck t = makeTypeCheck();
t.processForTesting(null, n);
return t.getTypedPercent();
}
private ObjectType getInstanceType(Node js1Node) {
JSType type = js1Node.getFirstChild().getJSType();
assertNotNull(type);
assertTrue(type instanceof FunctionType);
FunctionType functionType = (FunctionType) type;
assertTrue(functionType.isConstructor());
return functionType.getInstanceType();
}
public void testPrototypePropertyReference() throws Exception {
TypeCheckResult p = parseAndTypeCheckWithScope(""
+ "/** @constructor */\n"
+ "function Foo() {}\n"
+ "/** @param {number} a */\n"
+ "Foo.prototype.bar = function(a){};\n"
+ "/** @param {Foo} f */\n"
+ "function baz(f) {\n"
+ " Foo.prototype.bar.call(f, 3);\n"
+ "}");
assertEquals(0, compiler.getErrorCount());
assertEquals(0, compiler.getWarningCount());
assertTrue(p.scope.getVar("Foo").getType() instanceof FunctionType);
FunctionType fooType = (FunctionType) p.scope.getVar("Foo").getType();
assertEquals("function (this:Foo, number): undefined",
fooType.getPrototype().getPropertyType("bar").toString());
}
public void testResolvingNamedTypes() throws Exception {
String js = ""
+ "/** @constructor */\n"
+ "var Foo = function() {}\n"
+ "/** @param {number} a */\n"
+ "Foo.prototype.foo = function(a) {\n"
+ " return this.baz().toString();\n"
+ "};\n"
+ "/** @return {Baz} */\n"
+ "Foo.prototype.baz = function() { return new Baz(); };\n"
+ "/** @constructor\n"
+ " * @extends Foo */\n"
+ "var Bar = function() {};"
+ "/** @constructor */\n"
+ "var Baz = function() {};";
assertEquals(100.0, getTypedPercent(js), 0.1);
}
public void testMissingProperty1() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() { return this.a; };" +
"Foo.prototype.baz = function() { this.a = 3; };");
}
public void testMissingProperty2() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() { return this.a; };" +
"Foo.prototype.baz = function() { this.b = 3; };",
"Property a never defined on Foo");
}
public void testMissingProperty3() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() { return this.a; };" +
"(new Foo).a = 3;");
}
public void testMissingProperty4() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() { return this.a; };" +
"(new Foo).b = 3;",
"Property a never defined on Foo");
}
public void testMissingProperty5() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() { return this.a; };" +
"/** @constructor */ function Bar() { this.a = 3; };",
"Property a never defined on Foo");
}
public void testMissingProperty6() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() { return this.a; };" +
"/** @constructor \n * @extends {Foo} */ " +
"function Bar() { this.a = 3; };");
}
public void testMissingProperty7() throws Exception {
testTypes(
"/** @param {Object} obj */" +
"function foo(obj) { return obj.impossible; }",
"Property impossible never defined on Object");
}
public void testMissingProperty8() throws Exception {
testTypes(
"/** @param {Object} obj */" +
"function foo(obj) { return typeof obj.impossible; }");
}
public void testMissingProperty9() throws Exception {
testTypes(
"/** @param {Object} obj */" +
"function foo(obj) { if (obj.impossible) { return true; } }");
}
public void testMissingProperty10() throws Exception {
testTypes(
"/** @param {Object} obj */" +
"function foo(obj) { while (obj.impossible) { return true; } }");
}
public void testMissingProperty11() throws Exception {
testTypes(
"/** @param {Object} obj */" +
"function foo(obj) { for (;obj.impossible;) { return true; } }");
}
public void testMissingProperty12() throws Exception {
testTypes(
"/** @param {Object} obj */" +
"function foo(obj) { do { } while (obj.impossible); }");
}
public void testMissingProperty13() throws Exception {
testTypes(
"var goog = {}; goog.isDef = function(x) { return false; };" +
"/** @param {Object} obj */" +
"function foo(obj) { return goog.isDef(obj.impossible); }");
}
public void testMissingProperty14() throws Exception {
testTypes(
"var goog = {}; goog.isDef = function(x) { return false; };" +
"/** @param {Object} obj */" +
"function foo(obj) { return goog.isNull(obj.impossible); }",
"Property isNull never defined on goog");
}
public void testMissingProperty15() throws Exception {
testTypes(
"/** @param {Object} x */" +
"function f(x) { if (x.foo) { x.foo(); } }");
}
public void testMissingProperty16() throws Exception {
testTypes(
"/** @param {Object} x */" +
"function f(x) { x.foo(); if (x.foo) {} }",
"Property foo never defined on Object");
}
public void testMissingProperty17() throws Exception {
testTypes(
"/** @param {Object} x */" +
"function f(x) { if (typeof x.foo == 'function') { x.foo(); } }");
}
public void testMissingProperty18() throws Exception {
testTypes(
"/** @param {Object} x */" +
"function f(x) { if (x.foo instanceof Function) { x.foo(); } }");
}
public void testMissingProperty19() throws Exception {
testTypes(
"/** @param {Object} x */" +
"function f(x) { if (x.bar) { if (x.foo) {} } else { x.foo(); } }",
"Property foo never defined on Object");
}
public void testMissingProperty20() throws Exception {
testTypes(
"/** @param {Object} x */" +
"function f(x) { if (x.foo) { } else { x.foo(); } }",
"Property foo never defined on Object");
}
public void testMissingProperty21() throws Exception {
testTypes(
"/** @param {Object} x */" +
"function f(x) { x.foo && x.foo(); }");
}
public void testMissingProperty22() throws Exception {
testTypes(
"/** @param {Object} x \n * @return {boolean} */" +
"function f(x) { return x.foo ? x.foo() : true; }");
}
public void testMissingProperty23() throws Exception {
testTypes(
"function f(x) { x.impossible(); }",
"Property impossible never defined on x");
}
public void testMissingProperty24() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MissingType'], []);" +
"/** @param {MissingType} x */" +
"function f(x) { x.impossible(); }", null);
}
public void testMissingProperty25() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {};" +
"Foo.prototype.bar = function() {};" +
"/** @constructor */ var FooAlias = Foo;" +
"(new FooAlias()).bar();");
}
public void testMissingProperty26() throws Exception {
testTypes(
"/** @constructor */ var Foo = function() {};" +
"/** @constructor */ var FooAlias = Foo;" +
"FooAlias.prototype.bar = function() {};" +
"(new Foo()).bar();");
}
public void testMissingProperty27() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MissingType'], []);" +
"/** @param {?MissingType} x */" +
"function f(x) {" +
" for (var parent = x; parent; parent = parent.getParent()) {}" +
"}", null);
}
public void testMissingProperty28() throws Exception {
testTypes(
"function f(obj) {" +
" /** @type {*} */ obj.foo;" +
" return obj.foo;" +
"}");
testTypes(
"function f(obj) {" +
" /** @type {*} */ obj.foo;" +
" return obj.foox;" +
"}",
"Property foox never defined on obj");
}
public void testMissingProperty29() throws Exception {
// This used to emit a warning.
testTypes(
// externs
"/** @constructor */ var Foo;" +
"Foo.prototype.opera;" +
"Foo.prototype.opera.postError;",
"",
null,
false);
}
public void testMissingProperty30() throws Exception {
testTypes(
"/** @return {*} */" +
"function f() {" +
" return {};" +
"}" +
"f().a = 3;" +
"/** @param {Object} y */ function g(y) { return y.a; }");
}
public void testMissingProperty31() throws Exception {
testTypes(
"/** @return {Array|number} */" +
"function f() {" +
" return [];" +
"}" +
"f().a = 3;" +
"/** @param {Array} y */ function g(y) { return y.a; }");
}
public void testMissingProperty32() throws Exception {
testTypes(
"/** @return {Array|number} */" +
"function f() {" +
" return [];" +
"}" +
"f().a = 3;" +
"/** @param {Date} y */ function g(y) { return y.a; }",
"Property a never defined on Date");
}
public void testMissingProperty33() throws Exception {
testTypes(
"/** @param {Object} x */" +
"function f(x) { !x.foo || x.foo(); }");
}
public void testMissingProperty34() throws Exception {
testTypes(
"/** @fileoverview \n * @suppress {missingProperties} */" +
"/** @constructor */ function Foo() {}" +
"Foo.prototype.bar = function() { return this.a; };" +
"Foo.prototype.baz = function() { this.b = 3; };");
}
public void testMissingProperty35() throws Exception {
// Bar has specialProp defined, so Bar|Baz may have specialProp defined.
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @constructor */ function Bar() {}" +
"/** @constructor */ function Baz() {}" +
"/** @param {Foo|Bar} x */ function f(x) { x.specialProp = 1; }" +
"/** @param {Bar|Baz} x */ function g(x) { return x.specialProp; }");
}
public void testMissingProperty36() throws Exception {
// Foo has baz defined, and SubFoo has bar defined, so some objects with
// bar may have baz.
testTypes(
"/** @constructor */ function Foo() {}" +
"Foo.prototype.baz = 0;" +
"/** @constructor \n * @extends {Foo} */ function SubFoo() {}" +
"SubFoo.prototype.bar = 0;" +
"/** @param {{bar: number}} x */ function f(x) { return x.baz; }");
}
public void testMissingProperty37() throws Exception {
// This used to emit a missing property warning because we couldn't
// determine that the inf(Foo, {isVisible:boolean}) == SubFoo.
testTypes(
"/** @param {{isVisible: boolean}} x */ function f(x){" +
" x.isVisible = false;" +
"}" +
"/** @constructor */ function Foo() {}" +
"/**\n" +
" * @constructor \n" +
" * @extends {Foo}\n" +
" */ function SubFoo() {}" +
"/** @type {boolean} */ SubFoo.prototype.isVisible = true;" +
"/**\n" +
" * @param {Foo} x\n" +
" * @return {boolean}\n" +
" */\n" +
"function g(x) { return x.isVisible; }");
}
public void testMissingProperty38() throws Exception {
testTypes(
"/** @constructor */ function Foo() {}" +
"/** @constructor */ function Bar() {}" +
"/** @return {Foo|Bar} */ function f() { return new Foo(); }" +
"f().missing;",
"Property missing never defined on (Bar|Foo|null)");
}
public void testMissingProperty39() throws Exception {
testTypes(
"/** @return {string|number} */ function f() { return 3; }" +
"f().length;");
}
public void testMissingProperty40() throws Exception {
testClosureTypes(
"goog.addDependency('zzz.js', ['MissingType'], []);" +
"/** @param {(Array|MissingType)} x */" +
"function f(x) { x.impossible(); }", null);
}
public void testMissingProperty41() throws Exception {
testTypes(
"/** @param {(Array|Date)} x */" +
"function f(x) { if (x.impossible) x.impossible(); }");
}
public void testMissingProperty42() throws Exception {
testTypes(
"/** @param {Object} x */" +
"function f(x) { " +
" if (typeof x.impossible == 'undefined') throw Error();" +
" return x.impossible;" +
"}");
}
public void testReflectObject1() throws Exception {
testClosureTypes(
"var goog = {}; goog.reflect = {}; " +
"goog.reflect.object = function(x, y){};" +
"/** @constructor */ function A() {}" +
"goog.reflect.object(A, {x: 3});",
null);
}
public void testReflectObject2() throws Exception {
testClosureTypes(
"var goog = {}; goog.reflect = {}; " +
"goog.reflect.object = function(x, y){};" +
"/** @param {string} x */ function f(x) {}" +
"/** @constructor */ function A() {}" +
"goog.reflect.object(A, {x: f(1 + 1)});",
"actual parameter 1 of f does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testLends1() throws Exception {
testTypes(
"function extend(x, y) {}" +
"/** @constructor */ function Foo() {}" +
"extend(Foo, /** @lends */ ({bar: 1}));",
"Bad type annotation. missing object name in @lends tag");
}
public void testLends2() throws Exception {
testTypes(
"function extend(x, y) {}" +
"/** @constructor */ function Foo() {}" +
"extend(Foo, /** @lends {Foob} */ ({bar: 1}));",
"Variable Foob not declared before @lends annotation.");
}
public void testLends3() throws Exception {
testTypes(
"function extend(x, y) {}" +
"/** @constructor */ function Foo() {}" +
"extend(Foo, {bar: 1});" +
"alert(Foo.bar);",
"Property bar never defined on Foo");
}
public void testLends4() throws Exception {
testTypes(
"function extend(x, y) {}" +
"/** @constructor */ function Foo() {}" +
"extend(Foo, /** @lends {Foo} */ ({bar: 1}));" +
"alert(Foo.bar);");
}
public void testLends5() throws Exception {
testTypes(
"function extend(x, y) {}" +
"/** @constructor */ function Foo() {}" +
"extend(Foo, {bar: 1});" +
"alert((new Foo()).bar);",
"Property bar never defined on Foo");
}
public void testLends6() throws Exception {
testTypes(
"function extend(x, y) {}" +
"/** @constructor */ function Foo() {}" +
"extend(Foo, /** @lends {Foo.prototype} */ ({bar: 1}));" +
"alert((new Foo()).bar);");
}
public void testLends7() throws Exception {
testTypes(
"function extend(x, y) {}" +
"/** @constructor */ function Foo() {}" +
"extend(Foo, /** @lends {Foo.prototype|Foo} */ ({bar: 1}));",
"Bad type annotation. expected closing }");
}
public void testLends8() throws Exception {
testTypes(
"function extend(x, y) {}" +
"/** @type {number} */ var Foo = 3;" +
"extend(Foo, /** @lends {Foo} */ ({bar: 1}));",
"May only lend properties to object types. Foo has type number.");
}
public void testLends9() throws Exception {
testClosureTypesMultipleWarnings(
"function extend(x, y) {}" +
"/** @constructor */ function Foo() {}" +
"extend(Foo, /** @lends {!Foo} */ ({bar: 1}));",
Lists.newArrayList(
"Bad type annotation. expected closing }",
"Bad type annotation. missing object name in @lends tag"));
}
public void testLends10() throws Exception {
testTypes(
"function defineClass(x) { return function() {}; } " +
"/** @constructor */" +
"var Foo = defineClass(" +
" /** @lends {Foo.prototype} */ ({/** @type {number} */ bar: 1}));" +
"/** @return {string} */ function f() { return (new Foo()).bar; }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testLends11() throws Exception {
testTypes(
"function defineClass(x, y) { return function() {}; } " +
"/** @constructor */" +
"var Foo = function() {};" +
"/** @return {*} */ Foo.prototype.bar = function() { return 3; };" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */\n" +
"var SubFoo = defineClass(Foo, " +
" /** @lends {SubFoo.prototype} */ ({\n" +
" /** @return {number} */ bar: function() { return 3; }}));" +
"/** @return {string} */ function f() { return (new SubFoo()).bar(); }",
"inconsistent return type\n" +
"found : number\n" +
"required: string");
}
public void testDeclaredNativeTypeEquality() throws Exception {
Node n = parseAndTypeCheck("/** @constructor */ function Object() {};");
assertTypeEquals(registry.getNativeType(JSTypeNative.OBJECT_FUNCTION_TYPE),
n.getFirstChild().getJSType());
}
public void testUndefinedVar() throws Exception {
Node n = parseAndTypeCheck("var undefined;");
assertTypeEquals(registry.getNativeType(JSTypeNative.VOID_TYPE),
n.getFirstChild().getFirstChild().getJSType());
}
public void testFlowScopeBug1() throws Exception {
Node n = parseAndTypeCheck("/** @param {number} a \n"
+ "* @param {number} b */\n"
+ "function f(a, b) {\n"
+ "/** @type number */"
+ "var i = 0;"
+ "for (; (i + a) < b; ++i) {}}");
// check the type of the add node for i + f
assertTypeEquals(registry.getNativeType(JSTypeNative.NUMBER_TYPE),
n.getFirstChild().getLastChild().getLastChild().getFirstChild()
.getNext().getFirstChild().getJSType());
}
public void testFlowScopeBug2() throws Exception {
Node n = parseAndTypeCheck("/** @constructor */ function Foo() {};\n"
+ "Foo.prototype.hi = false;"
+ "function foo(a, b) {\n"
+ " /** @type Array */"
+ " var arr;"
+ " /** @type number */"
+ " var iter;"
+ " for (iter = 0; iter < arr.length; ++ iter) {"
+ " /** @type Foo */"
+ " var afoo = arr[iter];"
+ " afoo;"
+ " }"
+ "}");
// check the type of afoo when referenced
assertTypeEquals(registry.createNullableType(registry.getType("Foo")),
n.getLastChild().getLastChild().getLastChild().getLastChild()
.getLastChild().getLastChild().getJSType());
}
public void testAddSingletonGetter() {
Node n = parseAndTypeCheck(
"/** @constructor */ function Foo() {};\n" +
"goog.addSingletonGetter(Foo);");
ObjectType o = (ObjectType) n.getFirstChild().getJSType();
assertEquals("function (): Foo",
o.getPropertyType("getInstance").toString());
assertEquals("Foo", o.getPropertyType("instance_").toString());
}
public void testTypeCheckStandaloneAST() throws Exception {
Node n = compiler.parseTestCode("function Foo() { }");
typeCheck(n);
MemoizedScopeCreator scopeCreator = new MemoizedScopeCreator(
new TypedScopeCreator(compiler));
Scope topScope = scopeCreator.createScope(n, null);
Node second = compiler.parseTestCode("new Foo");
Node externs = new Node(Token.BLOCK);
Node externAndJsRoot = new Node(Token.BLOCK, externs, second);
externAndJsRoot.setIsSyntheticBlock(true);
new TypeCheck(
compiler,
new SemanticReverseAbstractInterpreter(
compiler.getCodingConvention(), registry),
registry, topScope, scopeCreator, CheckLevel.WARNING, CheckLevel.OFF)
.process(null, second);
assertEquals(1, compiler.getWarningCount());
assertEquals("cannot instantiate non-constructor",
compiler.getWarnings()[0].description);
}
public void testUpdateParameterTypeOnClosure() throws Exception {
testTypes(
"/**\n" +
"* @constructor\n" +
"* @param {*=} opt_value\n" +
"* @return {?}\n" +
"*/\n" +
"function Object(opt_value) {}\n" +
"/**\n" +
"* @constructor\n" +
"* @param {...*} var_args\n" +
"*/\n" +
"function Function(var_args) {}\n" +
"/**\n" +
"* @type {Function}\n" +
"*/\n" +
// The line below sets JSDocInfo on Object so that the type of the
// argument to function f has JSDoc through its prototype chain.
"Object.prototype.constructor = function() {};\n",
"/**\n" +
"* @param {function(): boolean} fn\n" +
"*/\n" +
"function f(fn) {}\n" +
"f(function(g) { });\n",
null,
false);
}
public void testTemplatedThisType1() throws Exception {
testTypes(
"/** @constructor */\n" +
"function Foo() {}\n" +
"/**\n" +
" * @this {T}\n" +
" * @return {T}\n" +
" * @template T\n" +
" */\n" +
"Foo.prototype.method = function() {};\n" +
"/**\n" +
" * @constructor\n" +
" * @extends {Foo}\n" +
" */\n" +
"function Bar() {}\n" +
"var g = new Bar().method();\n" +
"/**\n" +
" * @param {number} a\n" +
" */\n" +
"function compute(a) {};\n" +
"compute(g);\n",
"actual parameter 1 of compute does not match formal parameter\n" +
"found : Bar\n" +
"required: number");
}
public void testTemplatedThisType2() throws Exception {
testTypes(
"/**\n" +
" * @this {Array.<T>|{length:number}}\n" +
" * @return {T}\n" +
" * @template T\n" +
" */\n" +
"Array.prototype.method = function() {};\n" +
"(function(){\n" +
" Array.prototype.method.call(arguments);" +
"})();");
}
public void testTemplateType1() throws Exception {
testTypes(
"/**\n" +
"* @param {T} x\n" +
"* @param {T} y\n" +
"* @param {function(this:T, ...)} z\n" +
"* @template T\n" +
"*/\n" +
"function f(x, y, z) {}\n" +
"f(this, this, function() { this });");
}
public void testTemplateType2() throws Exception {
// "this" types need to be coerced for ES3 style function or left
// allow for ES5-strict methods.
testTypes(
"/**\n" +
"* @param {T} x\n" +
"* @param {function(this:T, ...)} y\n" +
"* @template T\n" +
"*/\n" +
"function f(x, y) {}\n" +
"f(0, function() {});");
}
public void testTemplateType3() throws Exception {
testTypes(
"/**" +
" * @param {T} v\n" +
" * @param {function(T)} f\n" +
" * @template T\n" +
" */\n" +
"function call(v, f) { f.call(null, v); }" +
"/** @type {string} */ var s;" +
"call(3, function(x) {" +
" x = true;" +
" s = x;" +
"});",
"assignment\n" +
"found : boolean\n" +
"required: string");
}
public void testTemplateType4() throws Exception {
testTypes(
"/**" +
" * @param {...T} p\n" +
" * @return {T} \n" +
" * @template T\n" +
" */\n" +
"function fn(p) { return p; }\n" +
"/** @type {!Object} */ var x;" +
"x = fn(3, null);",
"assignment\n" +
"found : (null|number)\n" +
"required: Object");
}
public void testTemplateType5() throws Exception {
testTypes(
"/**" +
" * @param {Array.<T>} arr \n" +
" * @param {?function(T)} f \n" +
" * @return {T} \n" +
" * @template T\n" +
" */\n" +
"function fn(arr, f) { return arr[0]; }\n" +
"/** @param {Array.<number>} arr */ function g(arr) {" +
" /** @type {!Object} */ var x = fn.call(null, arr, null);" +
"}",
"initializing variable\n" +
"found : number\n" +
"required: Object");
}
public void disable_testBadTemplateType4() throws Exception {
// TODO(johnlenz): Add a check for useless of template types.
// Unless there are at least two references to a Template type in
// a definition it isn't useful.
testTypes(
"/**\n" +
"* @template T\n" +
"*/\n" +
"function f() {}\n" +
"f();",
FunctionTypeBuilder.TEMPLATE_TYPE_EXPECTED.format());
}
public void disable_testBadTemplateType5() throws Exception {
// TODO(johnlenz): Add a check for useless of template types.
// Unless there are at least two references to a Template type in
// a definition it isn't useful.
testTypes(
"/**\n" +
"* @template T\n" +
"* @return {T}\n" +
"*/\n" +
"function f() {}\n" +
"f();",
FunctionTypeBuilder.TEMPLATE_TYPE_EXPECTED.format());
}
public void disable_testFunctionLiteralUndefinedThisArgument()
throws Exception {
// TODO(johnlenz): this was a weird error. We should add a general
// restriction on what is accepted for T. Something like:
// "@template T of {Object|string}" or some such.
testTypes(""
+ "/**\n"
+ " * @param {function(this:T, ...)?} fn\n"
+ " * @param {?T} opt_obj\n"
+ " * @template T\n"
+ " */\n"
+ "function baz(fn, opt_obj) {}\n"
+ "baz(function() { this; });",
"Function literal argument refers to undefined this argument");
}
public void testFunctionLiteralDefinedThisArgument() throws Exception {
testTypes(""
+ "/**\n"
+ " * @param {function(this:T, ...)?} fn\n"
+ " * @param {?T} opt_obj\n"
+ " * @template T\n"
+ " */\n"
+ "function baz(fn, opt_obj) {}\n"
+ "baz(function() { this; }, {});");
}
public void testFunctionLiteralDefinedThisArgument2() throws Exception {
testTypes(""
+ "/** @param {string} x */ function f(x) {}"
+ "/**\n"
+ " * @param {?function(this:T, ...)} fn\n"
+ " * @param {T=} opt_obj\n"
+ " * @template T\n"
+ " */\n"
+ "function baz(fn, opt_obj) {}\n"
+ "function g() { baz(function() { f(this.length); }, []); }",
"actual parameter 1 of f does not match formal parameter\n"
+ "found : number\n"
+ "required: string");
}
public void testFunctionLiteralUnreadNullThisArgument() throws Exception {
testTypes(""
+ "/**\n"
+ " * @param {function(this:T, ...)?} fn\n"
+ " * @param {?T} opt_obj\n"
+ " * @template T\n"
+ " */\n"
+ "function baz(fn, opt_obj) {}\n"
+ "baz(function() {}, null);");
}
public void testUnionTemplateThisType() throws Exception {
testTypes(
"/** @constructor */ function F() {}" +
"/** @return {F|Array} */ function g() { return []; }" +
"/** @param {F} x */ function h(x) { }" +
"/**\n" +
"* @param {T} x\n" +
"* @param {function(this:T, ...)} y\n" +
"* @template T\n" +
"*/\n" +
"function f(x, y) {}\n" +
"f(g(), function() { h(this); });",
"actual parameter 1 of h does not match formal parameter\n" +
"found : (Array|F|null)\n" +
"required: (F|null)");
}
public void testActiveXObject() throws Exception {
testTypes(
"/** @type {Object} */ var x = new ActiveXObject();" +
"/** @type { {impossibleProperty} } */ var y = new ActiveXObject();");
}
public void testRecordType1() throws Exception {
testTypes(
"/** @param {{prop: number}} x */" +
"function f(x) {}" +
"f({});",
"actual parameter 1 of f does not match formal parameter\n" +
"found : {prop: (number|undefined)}\n" +
"required: {prop: number}");
}
public void testRecordType2() throws Exception {
testTypes(
"/** @param {{prop: (number|undefined)}} x */" +
"function f(x) {}" +
"f({});");
}
public void testRecordType3() throws Exception {
testTypes(
"/** @param {{prop: number}} x */" +
"function f(x) {}" +
"f({prop: 'x'});",
"actual parameter 1 of f does not match formal parameter\n" +
"found : {prop: (number|string)}\n" +
"required: {prop: number}");
}
public void testRecordType4() throws Exception {
// Notice that we do not do flow-based inference on the object type:
// We don't try to prove that x.prop may not be string until x
// gets passed to g.
testClosureTypesMultipleWarnings(
"/** @param {{prop: (number|undefined)}} x */" +
"function f(x) {}" +
"/** @param {{prop: (string|undefined)}} x */" +
"function g(x) {}" +
"var x = {}; f(x); g(x);",
Lists.newArrayList(
"actual parameter 1 of f does not match formal parameter\n" +
"found : {prop: (number|string|undefined)}\n" +
"required: {prop: (number|undefined)}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : {prop: (number|string|undefined)}\n" +
"required: {prop: (string|undefined)}"));
}
public void testRecordType5() throws Exception {
testTypes(
"/** @param {{prop: (number|undefined)}} x */" +
"function f(x) {}" +
"/** @param {{otherProp: (string|undefined)}} x */" +
"function g(x) {}" +
"var x = {}; f(x); g(x);");
}
public void testRecordType6() throws Exception {
testTypes(
"/** @return {{prop: (number|undefined)}} x */" +
"function f() { return {}; }");
}
public void testRecordType7() throws Exception {
testTypes(
"/** @return {{prop: (number|undefined)}} x */" +
"function f() { var x = {}; g(x); return x; }" +
"/** @param {number} x */" +
"function g(x) {}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : {prop: (number|undefined)}\n" +
"required: number");
}
public void testRecordType8() throws Exception {
testTypes(
"/** @return {{prop: (number|string)}} x */" +
"function f() { var x = {prop: 3}; g(x.prop); return x; }" +
"/** @param {string} x */" +
"function g(x) {}",
"actual parameter 1 of g does not match formal parameter\n" +
"found : number\n" +
"required: string");
}
public void testDuplicateRecordFields1() throws Exception {
testTypes("/**"
+ "* @param {{x:string, x:number}} a"
+ "*/"
+ "function f(a) {};",
"Parse error. Duplicate record field x");
}
public void testDuplicateRecordFields2() throws Exception {
testTypes("/**"
+ "* @param {{name:string,number:x,number:y}} a"
+ " */"
+ "function f(a) {};",
new String[] {"Bad type annotation. Unknown type x",
"Parse error. Duplicate record field number",
"Bad type annotation. Unknown type y"});
}
public void testMultipleExtendsInterface1() throws Exception {
testTypes("/** @interface */ function base1() {}\n"
+ "/** @interface */ function base2() {}\n"
+ "/** @interface\n"
+ "* @extends {base1}\n"
+ "* @extends {base2}\n"
+ "*/\n"
+ "function derived() {}");
}
public void testMultipleExtendsInterface2() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @desc description */Int0.prototype.foo = function() {};" +
"/** @interface \n @extends {Int0} \n @extends {Int1} */" +
"function Int2() {};" +
"/** @constructor\n @implements {Int2} */function Foo() {};",
"property foo on interface Int0 is not implemented by type Foo");
}
public void testMultipleExtendsInterface3() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @desc description */Int1.prototype.foo = function() {};" +
"/** @interface \n @extends {Int0} \n @extends {Int1} */" +
"function Int2() {};" +
"/** @constructor\n @implements {Int2} */function Foo() {};",
"property foo on interface Int1 is not implemented by type Foo");
}
public void testMultipleExtendsInterface4() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @interface \n @extends {Int0} \n @extends {Int1} \n" +
" @extends {number} */" +
"function Int2() {};" +
"/** @constructor\n @implements {Int2} */function Foo() {};",
"Int2 @extends non-object type number");
}
public void testMultipleExtendsInterface5() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @constructor */function Int1() {};" +
"/** @desc description @ return {string} x */" +
"/** @interface \n @extends {Int0} \n @extends {Int1} */" +
"function Int2() {};",
"Int2 cannot extend this type; interfaces can only extend interfaces");
}
public void testMultipleExtendsInterface6() throws Exception {
testTypes(
"/** @interface */function Super1() {};" +
"/** @interface */function Super2() {};" +
"/** @param {number} bar */Super2.prototype.foo = function(bar) {};" +
"/** @interface\n @extends {Super1}\n " +
"@extends {Super2} */function Sub() {};" +
"/** @override\n @param {string} bar */Sub.prototype.foo =\n" +
"function(bar) {};",
"mismatch of the foo property type and the type of the property it " +
"overrides from superclass Super2\n" +
"original: function (this:Super2, number): undefined\n" +
"override: function (this:Sub, string): undefined");
}
public void testMultipleExtendsInterfaceAssignment() throws Exception {
testTypes("/** @interface */var I1 = function() {};\n" +
"/** @interface */ var I2 = function() {}\n" +
"/** @interface\n@extends {I1}\n@extends {I2}*/" +
"var I3 = function() {};\n" +
"/** @constructor\n@implements {I3}*/var T = function() {};\n" +
"var t = new T();\n" +
"/** @type {I1} */var i1 = t;\n" +
"/** @type {I2} */var i2 = t;\n" +
"/** @type {I3} */var i3 = t;\n" +
"i1 = i3;\n" +
"i2 = i3;\n");
}
public void testMultipleExtendsInterfaceParamPass() throws Exception {
testTypes("/** @interface */var I1 = function() {};\n" +
"/** @interface */ var I2 = function() {}\n" +
"/** @interface\n@extends {I1}\n@extends {I2}*/" +
"var I3 = function() {};\n" +
"/** @constructor\n@implements {I3}*/var T = function() {};\n" +
"var t = new T();\n" +
"/** @param x I1 \n@param y I2\n@param z I3*/function foo(x,y,z){};\n" +
"foo(t,t,t)\n");
}
public void testBadMultipleExtendsClass() throws Exception {
testTypes("/** @constructor */ function base1() {}\n"
+ "/** @constructor */ function base2() {}\n"
+ "/** @constructor\n"
+ "* @extends {base1}\n"
+ "* @extends {base2}\n"
+ "*/\n"
+ "function derived() {}",
"Bad type annotation. type annotation incompatible "
+ "with other annotations");
}
public void testInterfaceExtendsResolution() throws Exception {
testTypes("/** @interface \n @extends {A} */ function B() {};\n" +
"/** @constructor \n @implements {B} */ function C() {};\n" +
"/** @interface */ function A() {};");
}
public void testPropertyCanBeDefinedInObject() throws Exception {
testTypes("/** @interface */ function I() {};" +
"I.prototype.bar = function() {};" +
"/** @type {Object} */ var foo;" +
"foo.bar();");
}
private void checkObjectType(ObjectType objectType, String propertyName,
JSType expectedType) {
assertTrue("Expected " + objectType.getReferenceName() +
" to have property " +
propertyName, objectType.hasProperty(propertyName));
assertTypeEquals("Expected " + objectType.getReferenceName() +
"'s property " +
propertyName + " to have type " + expectedType,
expectedType, objectType.getPropertyType(propertyName));
}
public void testExtendedInterfacePropertiesCompatibility1() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @type {number} */" +
"Int0.prototype.foo;" +
"/** @type {string} */" +
"Int1.prototype.foo;" +
"/** @interface \n @extends {Int0} \n @extends {Int1} */" +
"function Int2() {};",
"Interface Int2 has a property foo with incompatible types in its " +
"super interfaces Int0 and Int1");
}
public void testExtendedInterfacePropertiesCompatibility2() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @interface */function Int2() {};" +
"/** @type {number} */" +
"Int0.prototype.foo;" +
"/** @type {string} */" +
"Int1.prototype.foo;" +
"/** @type {Object} */" +
"Int2.prototype.foo;" +
"/** @interface \n @extends {Int0} \n @extends {Int1} \n" +
"@extends {Int2}*/" +
"function Int3() {};",
new String[] {
"Interface Int3 has a property foo with incompatible types in " +
"its super interfaces Int0 and Int1",
"Interface Int3 has a property foo with incompatible types in " +
"its super interfaces Int1 and Int2"
});
}
public void testExtendedInterfacePropertiesCompatibility3() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @type {number} */" +
"Int0.prototype.foo;" +
"/** @type {string} */" +
"Int1.prototype.foo;" +
"/** @interface \n @extends {Int1} */ function Int2() {};" +
"/** @interface \n @extends {Int0} \n @extends {Int2} */" +
"function Int3() {};",
"Interface Int3 has a property foo with incompatible types in its " +
"super interfaces Int0 and Int1");
}
public void testExtendedInterfacePropertiesCompatibility4() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface \n @extends {Int0} */ function Int1() {};" +
"/** @type {number} */" +
"Int0.prototype.foo;" +
"/** @interface */function Int2() {};" +
"/** @interface \n @extends {Int2} */ function Int3() {};" +
"/** @type {string} */" +
"Int2.prototype.foo;" +
"/** @interface \n @extends {Int1} \n @extends {Int3} */" +
"function Int4() {};",
"Interface Int4 has a property foo with incompatible types in its " +
"super interfaces Int0 and Int2");
}
public void testExtendedInterfacePropertiesCompatibility5() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @type {number} */" +
"Int0.prototype.foo;" +
"/** @type {string} */" +
"Int1.prototype.foo;" +
"/** @interface \n @extends {Int1} */ function Int2() {};" +
"/** @interface \n @extends {Int0} \n @extends {Int2} */" +
"function Int3() {};" +
"/** @interface */function Int4() {};" +
"/** @type {number} */" +
"Int4.prototype.foo;" +
"/** @interface \n @extends {Int3} \n @extends {Int4} */" +
"function Int5() {};",
new String[] {
"Interface Int3 has a property foo with incompatible types in its" +
" super interfaces Int0 and Int1",
"Interface Int5 has a property foo with incompatible types in its" +
" super interfaces Int1 and Int4"});
}
public void testExtendedInterfacePropertiesCompatibility6() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @type {number} */" +
"Int0.prototype.foo;" +
"/** @type {string} */" +
"Int1.prototype.foo;" +
"/** @interface \n @extends {Int1} */ function Int2() {};" +
"/** @interface \n @extends {Int0} \n @extends {Int2} */" +
"function Int3() {};" +
"/** @interface */function Int4() {};" +
"/** @type {string} */" +
"Int4.prototype.foo;" +
"/** @interface \n @extends {Int3} \n @extends {Int4} */" +
"function Int5() {};",
"Interface Int3 has a property foo with incompatible types in its" +
" super interfaces Int0 and Int1");
}
public void testExtendedInterfacePropertiesCompatibility7() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @type {number} */" +
"Int0.prototype.foo;" +
"/** @type {string} */" +
"Int1.prototype.foo;" +
"/** @interface \n @extends {Int1} */ function Int2() {};" +
"/** @interface \n @extends {Int0} \n @extends {Int2} */" +
"function Int3() {};" +
"/** @interface */function Int4() {};" +
"/** @type {Object} */" +
"Int4.prototype.foo;" +
"/** @interface \n @extends {Int3} \n @extends {Int4} */" +
"function Int5() {};",
new String[] {
"Interface Int3 has a property foo with incompatible types in its" +
" super interfaces Int0 and Int1",
"Interface Int5 has a property foo with incompatible types in its" +
" super interfaces Int1 and Int4"});
}
public void testExtendedInterfacePropertiesCompatibility8() throws Exception {
testTypes(
"/** @interface */function Int0() {};" +
"/** @interface */function Int1() {};" +
"/** @type {number} */" +
"Int0.prototype.foo;" +
"/** @type {string} */" +
"Int1.prototype.bar;" +
"/** @interface \n @extends {Int1} */ function Int2() {};" +
"/** @interface \n @extends {Int0} \n @extends {Int2} */" +
"function Int3() {};" +
"/** @interface */function Int4() {};" +
"/** @type {Object} */" +
"Int4.prototype.foo;" +
"/** @type {Null} */" +
"Int4.prototype.bar;" +
"/** @interface \n @extends {Int3} \n @extends {Int4} */" +
"function Int5() {};",
new String[] {
"Interface Int5 has a property bar with incompatible types in its" +
" super interfaces Int1 and Int4",
"Interface Int5 has a property foo with incompatible types in its" +
" super interfaces Int0 and Int4"});
}
public void testGenerics1() throws Exception {
String FN_DECL = "/** \n" +
" * @param {T} x \n" +
" * @param {function(T):T} y \n" +
" * @template T\n" +
" */ \n" +
"function f(x,y) { return y(x); }\n";
testTypes(
FN_DECL +
"/** @type {string} */" +
"var out;" +
"/** @type {string} */" +
"var result = f('hi', function(x){ out = x; return x; });");
testTypes(
FN_DECL +
"/** @type {string} */" +
"var out;" +
"var result = f(0, function(x){ out = x; return x; });",
"assignment\n" +
"found : number\n" +
"required: string");
testTypes(
FN_DECL +
"var out;" +
"/** @type {string} */" +
"var result = f(0, function(x){ out = x; return x; });",
"assignment\n" +
"found : number\n" +
"required: string");
}
public void testFilter0()
throws Exception {
testTypes(
"/**\n" +
" * @param {T} arr\n" +
" * @return {T}\n" +
" * @template T\n" +
" */\n" +
"var filter = function(arr){};\n" +
"/** @type {!Array.<string>} */" +
"var arr;\n" +
"/** @type {!Array.<string>} */" +
"var result = filter(arr);");
}
public void testFilter1()
throws Exception {
testTypes(
"/**\n" +
" * @param {!Array.<T>} arr\n" +
" * @return {!Array.<T>}\n" +
" * @template T\n" +
" */\n" +
"var filter = function(arr){};\n" +
"/** @type {!Array.<string>} */" +
"var arr;\n" +
"/** @type {!Array.<string>} */" +
"var result = filter(arr);");
}
public void testFilter2()
throws Exception {
testTypes(
"/**\n" +
" * @param {!Array.<T>} arr\n" +
" * @return {!Array.<T>}\n" +
" * @template T\n" +
" */\n" +
"var filter = function(arr){};\n" +
"/** @type {!Array.<string>} */" +
"var arr;\n" +
"/** @type {!Array.<number>} */" +
"var result = filter(arr);",
"initializing variable\n" +
"found : Array.<string>\n" +
"required: Array.<number>");
}
public void testFilter3()
throws Exception {
testTypes(
"/**\n" +
" * @param {Array.<T>} arr\n" +
" * @return {Array.<T>}\n" +
" * @template T\n" +
" */\n" +
"var filter = function(arr){};\n" +
"/** @type {Array.<string>} */" +
"var arr;\n" +
"/** @type {Array.<number>} */" +
"var result = filter(arr);",
"initializing variable\n" +
"found : (Array.<string>|null)\n" +
"required: (Array.<number>|null)");
}
public void testBackwardsInferenceGoogArrayFilter1()
throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {Array.<string>} */" +
"var arr;\n" +
"/** @type {!Array.<number>} */" +
"var result = goog.array.filter(" +
" arr," +
" function(item,index,src) {return false;});",
"initializing variable\n" +
"found : Array.<string>\n" +
"required: Array.<number>");
}
public void testBackwardsInferenceGoogArrayFilter2() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {number} */" +
"var out;" +
"/** @type {Array.<string>} */" +
"var arr;\n" +
"var out4 = goog.array.filter(" +
" arr," +
" function(item,index,src) {out = item;});",
"assignment\n" +
"found : string\n" +
"required: number");
}
public void testBackwardsInferenceGoogArrayFilter3() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {string} */" +
"var out;" +
"/** @type {Array.<string>} */ var arr;\n" +
"var result = goog.array.filter(" +
" arr," +
" function(item,index,src) {out = index;});",
"assignment\n" +
"found : number\n" +
"required: string");
}
public void testBackwardsInferenceGoogArrayFilter4() throws Exception {
testClosureTypes(
CLOSURE_DEFS +
"/** @type {string} */" +
"var out;" +
"/** @type {Array.<string>} */ var arr;\n" +
"var out4 = goog.array.filter(" +
" arr," +
" function(item,index,srcArr) {out = srcArr;});",
"assignment\n" +
"found : (null|{length: number})\n" +
"required: string");
}
public void testCatchExpression1() throws Exception {
testTypes(
"function fn() {" +
" /** @type {number} */" +
" var out = 0;" +
" try {\n" +
" foo();\n" +
" } catch (/** @type {string} */ e) {\n" +
" out = e;" +
" }" +
"}\n",
"assignment\n" +
"found : string\n" +
"required: number");
}
public void testCatchExpression2() throws Exception {
testTypes(
"function fn() {" +
" /** @type {number} */" +
" var out = 0;" +
" /** @type {string} */" +
" var e;" +
" try {\n" +
" foo();\n" +
" } catch (e) {\n" +
" out = e;" +
" }" +
"}\n");
}
public void testParameterized1() throws Exception {
testTypes(
"/** @type {!Array.<string>} */" +
"var arr1 = [];\n" +
"/** @type {!Array.<number>} */" +
"var arr2 = [];\n" +
"arr1 = arr2;",
"assignment\n" +
"found : Array.<number>\n" +
"required: Array.<string>");
}
public void testParameterized2() throws Exception {
testTypes(
"/** @type {!Array.<string>} */" +
"var arr1 = /** @type {!Array.<number>} */([]);\n",
"initializing variable\n" +
"found : Array.<number>\n" +
"required: Array.<string>");
}
public void testParameterized3() throws Exception {
testTypes(
"/** @type {Array.<string>} */" +
"var arr1 = /** @type {!Array.<number>} */([]);\n",
"initializing variable\n" +
"found : Array.<number>\n" +
"required: (Array.<string>|null)");
}
public void testParameterized4() throws Exception {
testTypes(
"/** @type {Array.<string>} */" +
"var arr1 = [];\n" +
"/** @type {Array.<number>} */" +
"var arr2 = arr1;\n",
"initializing variable\n" +
"found : (Array.<string>|null)\n" +
"required: (Array.<number>|null)");
}
public void testParameterizedTypeSubtypes2() throws Exception {
JSType arrayOfNumber = createParameterizedType(
ARRAY_TYPE, NUMBER_TYPE);
JSType arrayOfString = createParameterizedType(
ARRAY_TYPE, STRING_TYPE);
assertFalse(arrayOfString.isSubtype(createUnionType(arrayOfNumber, NULL_VOID)));
}
private void testTypes(String js) throws Exception {
testTypes(js, (String) null);
}
private void testTypes(String js, String description) throws Exception {
testTypes(js, description, false);
}
private void testTypes(String js, DiagnosticType type) throws Exception {
testTypes(js, type.format(), false);
}
private void testClosureTypes(String js, String description)
throws Exception {
testClosureTypesMultipleWarnings(js,
description == null ? null : Lists.newArrayList(description));
}
private void testClosureTypesMultipleWarnings(
String js, List<String> descriptions) throws Exception {
Node n = compiler.parseTestCode(js);
Node externs = new Node(Token.BLOCK);
Node externAndJsRoot = new Node(Token.BLOCK, externs, n);
externAndJsRoot.setIsSyntheticBlock(true);
assertEquals("parsing error: " +
Joiner.on(", ").join(compiler.getErrors()),
0, compiler.getErrorCount());
// For processing goog.addDependency for forward typedefs.
new ProcessClosurePrimitives(compiler, null, CheckLevel.ERROR)
.process(null, n);
CodingConvention convention = compiler.getCodingConvention();
new TypeCheck(compiler,
new ClosureReverseAbstractInterpreter(
convention, registry).append(
new SemanticReverseAbstractInterpreter(
convention, registry))
.getFirst(),
registry)
.processForTesting(null, n);
assertEquals(
"unexpected error(s) : " +
Joiner.on(", ").join(compiler.getErrors()),
0, compiler.getErrorCount());
if (descriptions == null) {
assertEquals(
"unexpected warning(s) : " +
Joiner.on(", ").join(compiler.getWarnings()),
0, compiler.getWarningCount());
} else {
assertEquals(
"unexpected warning(s) : " +
Joiner.on(", ").join(compiler.getWarnings()),
descriptions.size(), compiler.getWarningCount());
Set<String> actualWarningDescriptions = Sets.newHashSet();
for (int i = 0; i < descriptions.size(); i++) {
actualWarningDescriptions.add(compiler.getWarnings()[i].description);
}
assertEquals(
Sets.newHashSet(descriptions), actualWarningDescriptions);
}
}
void testTypes(String js, String description, boolean isError)
throws Exception {
testTypes(DEFAULT_EXTERNS, js, description, isError);
}
void testTypes(String externs, String js, String description, boolean isError)
throws Exception {
Node n = parseAndTypeCheck(externs, js);
JSError[] errors = compiler.getErrors();
if (description != null && isError) {
assertTrue("expected an error", errors.length > 0);
assertEquals(description, errors[0].description);
errors = Arrays.asList(errors).subList(1, errors.length).toArray(
new JSError[errors.length - 1]);
}
if (errors.length > 0) {
fail("unexpected error(s):\n" + Joiner.on("\n").join(errors));
}
JSError[] warnings = compiler.getWarnings();
if (description != null && !isError) {
assertTrue("expected a warning", warnings.length > 0);
assertEquals(description, warnings[0].description);
warnings = Arrays.asList(warnings).subList(1, warnings.length).toArray(
new JSError[warnings.length - 1]);
}
if (warnings.length > 0) {
fail("unexpected warnings(s):\n" + Joiner.on("\n").join(warnings));
}
}
/**
* Parses and type checks the JavaScript code.
*/
private Node parseAndTypeCheck(String js) {
return parseAndTypeCheck(DEFAULT_EXTERNS, js);
}
private Node parseAndTypeCheck(String externs, String js) {
return parseAndTypeCheckWithScope(externs, js).root;
}
/**
* Parses and type checks the JavaScript code and returns the Scope used
* whilst type checking.
*/
private TypeCheckResult parseAndTypeCheckWithScope(String js) {
return parseAndTypeCheckWithScope(DEFAULT_EXTERNS, js);
}
private TypeCheckResult parseAndTypeCheckWithScope(
String externs, String js) {
compiler.init(
Lists.newArrayList(SourceFile.fromCode("[externs]", externs)),
Lists.newArrayList(SourceFile.fromCode("[testcode]", js)),
compiler.getOptions());
Node n = compiler.getInput(new InputId("[testcode]")).getAstRoot(compiler);
Node externsNode = compiler.getInput(new InputId("[externs]"))
.getAstRoot(compiler);
Node externAndJsRoot = new Node(Token.BLOCK, externsNode, n);
externAndJsRoot.setIsSyntheticBlock(true);
assertEquals("parsing error: " +
Joiner.on(", ").join(compiler.getErrors()),
0, compiler.getErrorCount());
Scope s = makeTypeCheck().processForTesting(externsNode, n);
return new TypeCheckResult(n, s);
}
private Node typeCheck(Node n) {
Node externsNode = new Node(Token.BLOCK);
Node externAndJsRoot = new Node(Token.BLOCK, externsNode, n);
externAndJsRoot.setIsSyntheticBlock(true);
makeTypeCheck().processForTesting(null, n);
return n;
}
private TypeCheck makeTypeCheck() {
return new TypeCheck(
compiler,
new SemanticReverseAbstractInterpreter(
compiler.getCodingConvention(), registry),
registry,
reportMissingOverrides,
CheckLevel.OFF);
}
void testTypes(String js, String[] warnings) throws Exception {
Node n = compiler.parseTestCode(js);
assertEquals(0, compiler.getErrorCount());
Node externsNode = new Node(Token.BLOCK);
Node externAndJsRoot = new Node(Token.BLOCK, externsNode, n);
makeTypeCheck().processForTesting(null, n);
assertEquals(0, compiler.getErrorCount());
if (warnings != null) {
assertEquals(warnings.length, compiler.getWarningCount());
JSError[] messages = compiler.getWarnings();
for (int i = 0; i < warnings.length && i < compiler.getWarningCount();
i++) {
assertEquals(warnings[i], messages[i].description);
}
} else {
assertEquals(0, compiler.getWarningCount());
}
}
String suppressMissingProperty(String ... props) {
String result = "function dummy(x) { ";
for (String prop : props) {
result += "x." + prop + " = 3;";
}
return result + "}";
}
private static class TypeCheckResult {
private final Node root;
private final Scope scope;
private TypeCheckResult(Node root, Scope scope) {
this.root = root;
this.scope = scope;
}
}
} |
||
public void testFileValidator() {
final DefaultOptionBuilder obuilder = new DefaultOptionBuilder();
final ArgumentBuilder abuilder = new ArgumentBuilder();
final GroupBuilder gbuilder = new GroupBuilder();
DefaultOption fileNameOption = obuilder.withShortName("f")
.withLongName("file-name").withRequired(true).withDescription(
"name of an existing file").withArgument(
abuilder.withName("file-name").withValidator(
FileValidator.getExistingFileInstance())
.create()).create();
Group options = gbuilder.withName("options").withOption(fileNameOption)
.create();
Parser parser = new Parser();
parser.setHelpTrigger("--help");
parser.setGroup(options);
final String fileName = "src/test/org/apache/commons/cli2/bug/BugCLI144Test.java";
CommandLine cl = parser
.parseAndHelp(new String[] { "--file-name", fileName });
assertNotNull(cl);
assertEquals("Wrong file", new File(fileName), cl.getValue(fileNameOption));
} | org.apache.commons.cli2.bug.BugCLI144Test::testFileValidator | src/test/org/apache/commons/cli2/bug/BugCLI144Test.java | 64 | src/test/org/apache/commons/cli2/bug/BugCLI144Test.java | testFileValidator | /**
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.cli2.bug;
import java.io.File;
import junit.framework.TestCase;
import org.apache.commons.cli2.CommandLine;
import org.apache.commons.cli2.Group;
import org.apache.commons.cli2.builder.ArgumentBuilder;
import org.apache.commons.cli2.builder.DefaultOptionBuilder;
import org.apache.commons.cli2.builder.GroupBuilder;
import org.apache.commons.cli2.commandline.Parser;
import org.apache.commons.cli2.option.DefaultOption;
import org.apache.commons.cli2.validation.FileValidator;
/**
* Test case for http://issues.apache.org/jira/browse/CLI-144 CLI 2 throws
*
* <pre>
* Exception in thread "main" java.lang.ClassCastException: java.io.File cannot be cast to java.lang.String
* </pre>
*
* when there should be no such exception
*
* @author David Biesack
*/
public class BugCLI144Test extends TestCase {
public void testFileValidator() {
final DefaultOptionBuilder obuilder = new DefaultOptionBuilder();
final ArgumentBuilder abuilder = new ArgumentBuilder();
final GroupBuilder gbuilder = new GroupBuilder();
DefaultOption fileNameOption = obuilder.withShortName("f")
.withLongName("file-name").withRequired(true).withDescription(
"name of an existing file").withArgument(
abuilder.withName("file-name").withValidator(
FileValidator.getExistingFileInstance())
.create()).create();
Group options = gbuilder.withName("options").withOption(fileNameOption)
.create();
Parser parser = new Parser();
parser.setHelpTrigger("--help");
parser.setGroup(options);
final String fileName = "src/test/org/apache/commons/cli2/bug/BugCLI144Test.java";
CommandLine cl = parser
.parseAndHelp(new String[] { "--file-name", fileName });
assertNotNull(cl);
assertEquals("Wrong file", new File(fileName), cl.getValue(fileNameOption));
}
} | // You are a professional Java test case writer, please create a test case named `testFileValidator` for the issue `Cli-CLI-144`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Cli-CLI-144
//
// ## Issue-Title:
// adding a FileValidator results in ClassCastException in parser.parseAndHelp(args)
//
// ## Issue-Description:
//
// When I add a FileValidator.getExistingFileInstance() to an Argument, I get a ClassCastException when I parse args.
//
//
// Below is a testcase invoke with
//
//
// java org.apache.commons.cli2.issues.CLI2Sample -classpath commons-cli-2.0-SNAPSHOT.jar --file-name path-to-an-existing-file
//
//
// Run it and you get:
//
//
// Exception in thread "main" java.lang.ClassCastException: java.io.File cannot be cast to java.lang.String
//
// at org.apache.commons.cli2.validation.FileValidator.validate(FileValidator.java:122)
//
// at org.apache.commons.cli2.option.ArgumentImpl.validate(ArgumentImpl.java:250)
//
// at org.apache.commons.cli2.option.ParentImpl.validate(ParentImpl.java:123)
//
// at org.apache.commons.cli2.option.DefaultOption.validate(DefaultOption.java:175)
//
// at org.apache.commons.cli2.option.GroupImpl.validate(GroupImpl.java:264)
//
// at org.apache.commons.cli2.commandline.Parser.parse(Parser.java:105)
//
// at org.apache.commons.cli2.commandline.Parser.parseAndHelp(Parser.java:125)
//
// at org.apache.commons.cli2.issues.CLI2Sample.main(CLI2Sample.java:38)
//
//
// Comment out the withValidator call and it runs with no exception.
//
//
// I also get a similar ClassCastException if I add a
//
//
// .withValidator(NumberValidator.getIntegerInstance())
//
//
// to another option/argument.
//
//
// Here is the source
//
//
// package org.apache.commons.cli2.issues;
//
//
// import java.io.File;
//
// import org.apache.commons.cli2.CommandLine;
//
// import org.apache.commons.cli2.Group;
//
// import org.apache.commons.cli2.builder.ArgumentBuilder;
//
// import org.apache.commons.cli2.builder.DefaultOptionBuilder;
//
// import org.apache.commons.cli2.builder.GroupBuilder;
//
// import org.apache.commons.cli2.commandline.Parser;
//
// import org.apache.commons.cli2.option.DefaultOption;
//
// import org.apache.commons.cli2.validation.FileValidator;
//
//
// public class CLI2Sample
//
// {
//
// public static void main(String[] args)
//
//
// {
// final DefaultOptionBuilder obuilder = new DefaultOptionBuilder();
// final ArgumentBuilder abuilder = new ArgumentBuilder();
// final GroupBuilder gbuilder = new GroupBuilder();
// DefaultOption fileNameOption = obuilder
// .withShortName("f")
// .withLongName("file-name")
// .withRequired(true)
// .withDescription("name of an existing file")
// .withArgument(abuilder
// .withName("file-name")
// .withValidator(FileValidator.getExistingFileInstance())
// .create())
// .create();
// Group options = gbuilder
// .withName("options")
// .withOption(fileNameOption)
// .create();
// Parser parser = new Parser();
// parser.setHelpTrigger("--help");
// parser.setGroup(options);
// CommandLine cl = parser.parseAndHelp(args);
// }
// }
//
//
//
//
//
public void testFileValidator() {
| 64 | 14 | 43 | src/test/org/apache/commons/cli2/bug/BugCLI144Test.java | src/test | ```markdown
## Issue-ID: Cli-CLI-144
## Issue-Title:
adding a FileValidator results in ClassCastException in parser.parseAndHelp(args)
## Issue-Description:
When I add a FileValidator.getExistingFileInstance() to an Argument, I get a ClassCastException when I parse args.
Below is a testcase invoke with
java org.apache.commons.cli2.issues.CLI2Sample -classpath commons-cli-2.0-SNAPSHOT.jar --file-name path-to-an-existing-file
Run it and you get:
Exception in thread "main" java.lang.ClassCastException: java.io.File cannot be cast to java.lang.String
at org.apache.commons.cli2.validation.FileValidator.validate(FileValidator.java:122)
at org.apache.commons.cli2.option.ArgumentImpl.validate(ArgumentImpl.java:250)
at org.apache.commons.cli2.option.ParentImpl.validate(ParentImpl.java:123)
at org.apache.commons.cli2.option.DefaultOption.validate(DefaultOption.java:175)
at org.apache.commons.cli2.option.GroupImpl.validate(GroupImpl.java:264)
at org.apache.commons.cli2.commandline.Parser.parse(Parser.java:105)
at org.apache.commons.cli2.commandline.Parser.parseAndHelp(Parser.java:125)
at org.apache.commons.cli2.issues.CLI2Sample.main(CLI2Sample.java:38)
Comment out the withValidator call and it runs with no exception.
I also get a similar ClassCastException if I add a
.withValidator(NumberValidator.getIntegerInstance())
to another option/argument.
Here is the source
package org.apache.commons.cli2.issues;
import java.io.File;
import org.apache.commons.cli2.CommandLine;
import org.apache.commons.cli2.Group;
import org.apache.commons.cli2.builder.ArgumentBuilder;
import org.apache.commons.cli2.builder.DefaultOptionBuilder;
import org.apache.commons.cli2.builder.GroupBuilder;
import org.apache.commons.cli2.commandline.Parser;
import org.apache.commons.cli2.option.DefaultOption;
import org.apache.commons.cli2.validation.FileValidator;
public class CLI2Sample
{
public static void main(String[] args)
{
final DefaultOptionBuilder obuilder = new DefaultOptionBuilder();
final ArgumentBuilder abuilder = new ArgumentBuilder();
final GroupBuilder gbuilder = new GroupBuilder();
DefaultOption fileNameOption = obuilder
.withShortName("f")
.withLongName("file-name")
.withRequired(true)
.withDescription("name of an existing file")
.withArgument(abuilder
.withName("file-name")
.withValidator(FileValidator.getExistingFileInstance())
.create())
.create();
Group options = gbuilder
.withName("options")
.withOption(fileNameOption)
.create();
Parser parser = new Parser();
parser.setHelpTrigger("--help");
parser.setGroup(options);
CommandLine cl = parser.parseAndHelp(args);
}
}
```
You are a professional Java test case writer, please create a test case named `testFileValidator` for the issue `Cli-CLI-144`, utilizing the provided issue report information and the following function signature.
```java
public void testFileValidator() {
```
| 43 | [
"org.apache.commons.cli2.option.GroupImpl"
] | 9af16a9a4df4dd615bb6ff5832ac41b576e825199fea5aeef10d2688431b2f1e | public void testFileValidator() | // You are a professional Java test case writer, please create a test case named `testFileValidator` for the issue `Cli-CLI-144`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Cli-CLI-144
//
// ## Issue-Title:
// adding a FileValidator results in ClassCastException in parser.parseAndHelp(args)
//
// ## Issue-Description:
//
// When I add a FileValidator.getExistingFileInstance() to an Argument, I get a ClassCastException when I parse args.
//
//
// Below is a testcase invoke with
//
//
// java org.apache.commons.cli2.issues.CLI2Sample -classpath commons-cli-2.0-SNAPSHOT.jar --file-name path-to-an-existing-file
//
//
// Run it and you get:
//
//
// Exception in thread "main" java.lang.ClassCastException: java.io.File cannot be cast to java.lang.String
//
// at org.apache.commons.cli2.validation.FileValidator.validate(FileValidator.java:122)
//
// at org.apache.commons.cli2.option.ArgumentImpl.validate(ArgumentImpl.java:250)
//
// at org.apache.commons.cli2.option.ParentImpl.validate(ParentImpl.java:123)
//
// at org.apache.commons.cli2.option.DefaultOption.validate(DefaultOption.java:175)
//
// at org.apache.commons.cli2.option.GroupImpl.validate(GroupImpl.java:264)
//
// at org.apache.commons.cli2.commandline.Parser.parse(Parser.java:105)
//
// at org.apache.commons.cli2.commandline.Parser.parseAndHelp(Parser.java:125)
//
// at org.apache.commons.cli2.issues.CLI2Sample.main(CLI2Sample.java:38)
//
//
// Comment out the withValidator call and it runs with no exception.
//
//
// I also get a similar ClassCastException if I add a
//
//
// .withValidator(NumberValidator.getIntegerInstance())
//
//
// to another option/argument.
//
//
// Here is the source
//
//
// package org.apache.commons.cli2.issues;
//
//
// import java.io.File;
//
// import org.apache.commons.cli2.CommandLine;
//
// import org.apache.commons.cli2.Group;
//
// import org.apache.commons.cli2.builder.ArgumentBuilder;
//
// import org.apache.commons.cli2.builder.DefaultOptionBuilder;
//
// import org.apache.commons.cli2.builder.GroupBuilder;
//
// import org.apache.commons.cli2.commandline.Parser;
//
// import org.apache.commons.cli2.option.DefaultOption;
//
// import org.apache.commons.cli2.validation.FileValidator;
//
//
// public class CLI2Sample
//
// {
//
// public static void main(String[] args)
//
//
// {
// final DefaultOptionBuilder obuilder = new DefaultOptionBuilder();
// final ArgumentBuilder abuilder = new ArgumentBuilder();
// final GroupBuilder gbuilder = new GroupBuilder();
// DefaultOption fileNameOption = obuilder
// .withShortName("f")
// .withLongName("file-name")
// .withRequired(true)
// .withDescription("name of an existing file")
// .withArgument(abuilder
// .withName("file-name")
// .withValidator(FileValidator.getExistingFileInstance())
// .create())
// .create();
// Group options = gbuilder
// .withName("options")
// .withOption(fileNameOption)
// .create();
// Parser parser = new Parser();
// parser.setHelpTrigger("--help");
// parser.setGroup(options);
// CommandLine cl = parser.parseAndHelp(args);
// }
// }
//
//
//
//
//
| Cli | /**
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.cli2.bug;
import java.io.File;
import junit.framework.TestCase;
import org.apache.commons.cli2.CommandLine;
import org.apache.commons.cli2.Group;
import org.apache.commons.cli2.builder.ArgumentBuilder;
import org.apache.commons.cli2.builder.DefaultOptionBuilder;
import org.apache.commons.cli2.builder.GroupBuilder;
import org.apache.commons.cli2.commandline.Parser;
import org.apache.commons.cli2.option.DefaultOption;
import org.apache.commons.cli2.validation.FileValidator;
/**
* Test case for http://issues.apache.org/jira/browse/CLI-144 CLI 2 throws
*
* <pre>
* Exception in thread "main" java.lang.ClassCastException: java.io.File cannot be cast to java.lang.String
* </pre>
*
* when there should be no such exception
*
* @author David Biesack
*/
public class BugCLI144Test extends TestCase {
public void testFileValidator() {
final DefaultOptionBuilder obuilder = new DefaultOptionBuilder();
final ArgumentBuilder abuilder = new ArgumentBuilder();
final GroupBuilder gbuilder = new GroupBuilder();
DefaultOption fileNameOption = obuilder.withShortName("f")
.withLongName("file-name").withRequired(true).withDescription(
"name of an existing file").withArgument(
abuilder.withName("file-name").withValidator(
FileValidator.getExistingFileInstance())
.create()).create();
Group options = gbuilder.withName("options").withOption(fileNameOption)
.create();
Parser parser = new Parser();
parser.setHelpTrigger("--help");
parser.setGroup(options);
final String fileName = "src/test/org/apache/commons/cli2/bug/BugCLI144Test.java";
CommandLine cl = parser
.parseAndHelp(new String[] { "--file-name", fileName });
assertNotNull(cl);
assertEquals("Wrong file", new File(fileName), cl.getValue(fileNameOption));
}
} |
||
@Test
public void testMath713NegativeVariable() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] {1.0, 1.0}, 0.0d);
ArrayList<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] {1, 0}, Relationship.EQ, 1));
double epsilon = 1e-6;
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MINIMIZE, true);
Assert.assertTrue(Precision.compareTo(solution.getPoint()[0], 0.0d, epsilon) >= 0);
Assert.assertTrue(Precision.compareTo(solution.getPoint()[1], 0.0d, epsilon) >= 0);
} | org.apache.commons.math.optimization.linear.SimplexSolverTest::testMath713NegativeVariable | src/test/java/org/apache/commons/math/optimization/linear/SimplexSolverTest.java | 43 | src/test/java/org/apache/commons/math/optimization/linear/SimplexSolverTest.java | testMath713NegativeVariable | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.math.optimization.linear;
import org.junit.Assert;
import java.util.ArrayList;
import java.util.Collection;
import org.apache.commons.math.optimization.GoalType;
import org.apache.commons.math.optimization.RealPointValuePair;
import org.apache.commons.math.util.Precision;
import org.junit.Test;
public class SimplexSolverTest {
@Test
public void testMath713NegativeVariable() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] {1.0, 1.0}, 0.0d);
ArrayList<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] {1, 0}, Relationship.EQ, 1));
double epsilon = 1e-6;
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MINIMIZE, true);
Assert.assertTrue(Precision.compareTo(solution.getPoint()[0], 0.0d, epsilon) >= 0);
Assert.assertTrue(Precision.compareTo(solution.getPoint()[1], 0.0d, epsilon) >= 0);
}
@Test
public void testMath434NegativeVariable() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] {0.0, 0.0, 1.0}, 0.0d);
ArrayList<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] {1, 1, 0}, Relationship.EQ, 5));
constraints.add(new LinearConstraint(new double[] {0, 0, 1}, Relationship.GEQ, -10));
double epsilon = 1e-6;
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MINIMIZE, false);
Assert.assertEquals(5.0, solution.getPoint()[0] + solution.getPoint()[1], epsilon);
Assert.assertEquals(-10.0, solution.getPoint()[2], epsilon);
Assert.assertEquals(-10.0, solution.getValue(), epsilon);
}
@Test(expected = NoFeasibleSolutionException.class)
public void testMath434UnfeasibleSolution() {
double epsilon = 1e-6;
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] {1.0, 0.0}, 0.0);
ArrayList<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] {epsilon/2, 0.5}, Relationship.EQ, 0));
constraints.add(new LinearConstraint(new double[] {1e-3, 0.1}, Relationship.EQ, 10));
SimplexSolver solver = new SimplexSolver();
// allowing only non-negative values, no feasible solution shall be found
solver.optimize(f, constraints, GoalType.MINIMIZE, true);
}
@Test
public void testMath434PivotRowSelection() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] {1.0}, 0.0);
double epsilon = 1e-6;
ArrayList<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] {200}, Relationship.GEQ, 1));
constraints.add(new LinearConstraint(new double[] {100}, Relationship.GEQ, 0.499900001));
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MINIMIZE, false);
Assert.assertTrue(Precision.compareTo(solution.getPoint()[0] * 200.d, 1.d, epsilon) >= 0);
Assert.assertEquals(0.0050, solution.getValue(), epsilon);
}
@Test
public void testMath434PivotRowSelection2() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] {0.0d, 1.0d, 1.0d, 0.0d, 0.0d, 0.0d, 0.0d}, 0.0d);
ArrayList<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] {1.0d, -0.1d, 0.0d, 0.0d, 0.0d, 0.0d, 0.0d}, Relationship.EQ, -0.1d));
constraints.add(new LinearConstraint(new double[] {1.0d, 0.0d, 0.0d, 0.0d, 0.0d, 0.0d, 0.0d}, Relationship.GEQ, -1e-18d));
constraints.add(new LinearConstraint(new double[] {0.0d, 1.0d, 0.0d, 0.0d, 0.0d, 0.0d, 0.0d}, Relationship.GEQ, 0.0d));
constraints.add(new LinearConstraint(new double[] {0.0d, 0.0d, 0.0d, 1.0d, 0.0d, -0.0128588d, 1e-5d}, Relationship.EQ, 0.0d));
constraints.add(new LinearConstraint(new double[] {0.0d, 0.0d, 0.0d, 0.0d, 1.0d, 1e-5d, -0.0128586d}, Relationship.EQ, 1e-10d));
constraints.add(new LinearConstraint(new double[] {0.0d, 0.0d, 1.0d, -1.0d, 0.0d, 0.0d, 0.0d}, Relationship.GEQ, 0.0d));
constraints.add(new LinearConstraint(new double[] {0.0d, 0.0d, 1.0d, 1.0d, 0.0d, 0.0d, 0.0d}, Relationship.GEQ, 0.0d));
constraints.add(new LinearConstraint(new double[] {0.0d, 0.0d, 1.0d, 0.0d, -1.0d, 0.0d, 0.0d}, Relationship.GEQ, 0.0d));
constraints.add(new LinearConstraint(new double[] {0.0d, 0.0d, 1.0d, 0.0d, 1.0d, 0.0d, 0.0d}, Relationship.GEQ, 0.0d));
double epsilon = 1e-7;
SimplexSolver simplex = new SimplexSolver();
RealPointValuePair solution = simplex.optimize(f, constraints, GoalType.MINIMIZE, false);
Assert.assertTrue(Precision.compareTo(solution.getPoint()[0], -1e-18d, epsilon) >= 0);
Assert.assertEquals(1.0d, solution.getPoint()[1], epsilon);
Assert.assertEquals(0.0d, solution.getPoint()[2], epsilon);
Assert.assertEquals(1.0d, solution.getValue(), epsilon);
}
@Test
public void testMath272() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 2, 2, 1 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 1, 0 }, Relationship.GEQ, 1));
constraints.add(new LinearConstraint(new double[] { 1, 0, 1 }, Relationship.GEQ, 1));
constraints.add(new LinearConstraint(new double[] { 0, 1, 0 }, Relationship.GEQ, 1));
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MINIMIZE, true);
Assert.assertEquals(0.0, solution.getPoint()[0], .0000001);
Assert.assertEquals(1.0, solution.getPoint()[1], .0000001);
Assert.assertEquals(1.0, solution.getPoint()[2], .0000001);
Assert.assertEquals(3.0, solution.getValue(), .0000001);
}
@Test
public void testMath286() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 0.8, 0.2, 0.7, 0.3, 0.6, 0.4 }, 0 );
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 0, 1, 0, 1, 0 }, Relationship.EQ, 23.0));
constraints.add(new LinearConstraint(new double[] { 0, 1, 0, 1, 0, 1 }, Relationship.EQ, 23.0));
constraints.add(new LinearConstraint(new double[] { 1, 0, 0, 0, 0, 0 }, Relationship.GEQ, 10.0));
constraints.add(new LinearConstraint(new double[] { 0, 0, 1, 0, 0, 0 }, Relationship.GEQ, 8.0));
constraints.add(new LinearConstraint(new double[] { 0, 0, 0, 0, 1, 0 }, Relationship.GEQ, 5.0));
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, true);
Assert.assertEquals(25.8, solution.getValue(), .0000001);
Assert.assertEquals(23.0, solution.getPoint()[0] + solution.getPoint()[2] + solution.getPoint()[4], 0.0000001);
Assert.assertEquals(23.0, solution.getPoint()[1] + solution.getPoint()[3] + solution.getPoint()[5], 0.0000001);
Assert.assertTrue(solution.getPoint()[0] >= 10.0 - 0.0000001);
Assert.assertTrue(solution.getPoint()[2] >= 8.0 - 0.0000001);
Assert.assertTrue(solution.getPoint()[4] >= 5.0 - 0.0000001);
}
@Test
public void testDegeneracy() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 0.8, 0.7 }, 0 );
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 1 }, Relationship.LEQ, 18.0));
constraints.add(new LinearConstraint(new double[] { 1, 0 }, Relationship.GEQ, 10.0));
constraints.add(new LinearConstraint(new double[] { 0, 1 }, Relationship.GEQ, 8.0));
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, true);
Assert.assertEquals(13.6, solution.getValue(), .0000001);
}
@Test
public void testMath288() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 7, 3, 0, 0 }, 0 );
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 3, 0, -5, 0 }, Relationship.LEQ, 0.0));
constraints.add(new LinearConstraint(new double[] { 2, 0, 0, -5 }, Relationship.LEQ, 0.0));
constraints.add(new LinearConstraint(new double[] { 0, 3, 0, -5 }, Relationship.LEQ, 0.0));
constraints.add(new LinearConstraint(new double[] { 1, 0, 0, 0 }, Relationship.LEQ, 1.0));
constraints.add(new LinearConstraint(new double[] { 0, 1, 0, 0 }, Relationship.LEQ, 1.0));
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, true);
Assert.assertEquals(10.0, solution.getValue(), .0000001);
}
@Test
public void testMath290GEQ() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 1, 5 }, 0 );
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 2, 0 }, Relationship.GEQ, -1.0));
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MINIMIZE, true);
Assert.assertEquals(0, solution.getValue(), .0000001);
Assert.assertEquals(0, solution.getPoint()[0], .0000001);
Assert.assertEquals(0, solution.getPoint()[1], .0000001);
}
@Test(expected=NoFeasibleSolutionException.class)
public void testMath290LEQ() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 1, 5 }, 0 );
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 2, 0 }, Relationship.LEQ, -1.0));
SimplexSolver solver = new SimplexSolver();
solver.optimize(f, constraints, GoalType.MINIMIZE, true);
}
@Test
public void testMath293() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 0.8, 0.2, 0.7, 0.3, 0.4, 0.6}, 0 );
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 0, 1, 0, 1, 0 }, Relationship.EQ, 30.0));
constraints.add(new LinearConstraint(new double[] { 0, 1, 0, 1, 0, 1 }, Relationship.EQ, 30.0));
constraints.add(new LinearConstraint(new double[] { 0.8, 0.2, 0.0, 0.0, 0.0, 0.0 }, Relationship.GEQ, 10.0));
constraints.add(new LinearConstraint(new double[] { 0.0, 0.0, 0.7, 0.3, 0.0, 0.0 }, Relationship.GEQ, 10.0));
constraints.add(new LinearConstraint(new double[] { 0.0, 0.0, 0.0, 0.0, 0.4, 0.6 }, Relationship.GEQ, 10.0));
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution1 = solver.optimize(f, constraints, GoalType.MAXIMIZE, true);
Assert.assertEquals(15.7143, solution1.getPoint()[0], .0001);
Assert.assertEquals(0.0, solution1.getPoint()[1], .0001);
Assert.assertEquals(14.2857, solution1.getPoint()[2], .0001);
Assert.assertEquals(0.0, solution1.getPoint()[3], .0001);
Assert.assertEquals(0.0, solution1.getPoint()[4], .0001);
Assert.assertEquals(30.0, solution1.getPoint()[5], .0001);
Assert.assertEquals(40.57143, solution1.getValue(), .0001);
double valA = 0.8 * solution1.getPoint()[0] + 0.2 * solution1.getPoint()[1];
double valB = 0.7 * solution1.getPoint()[2] + 0.3 * solution1.getPoint()[3];
double valC = 0.4 * solution1.getPoint()[4] + 0.6 * solution1.getPoint()[5];
f = new LinearObjectiveFunction(new double[] { 0.8, 0.2, 0.7, 0.3, 0.4, 0.6}, 0 );
constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 0, 1, 0, 1, 0 }, Relationship.EQ, 30.0));
constraints.add(new LinearConstraint(new double[] { 0, 1, 0, 1, 0, 1 }, Relationship.EQ, 30.0));
constraints.add(new LinearConstraint(new double[] { 0.8, 0.2, 0.0, 0.0, 0.0, 0.0 }, Relationship.GEQ, valA));
constraints.add(new LinearConstraint(new double[] { 0.0, 0.0, 0.7, 0.3, 0.0, 0.0 }, Relationship.GEQ, valB));
constraints.add(new LinearConstraint(new double[] { 0.0, 0.0, 0.0, 0.0, 0.4, 0.6 }, Relationship.GEQ, valC));
RealPointValuePair solution2 = solver.optimize(f, constraints, GoalType.MAXIMIZE, true);
Assert.assertEquals(40.57143, solution2.getValue(), .0001);
}
@Test
public void testSimplexSolver() {
LinearObjectiveFunction f =
new LinearObjectiveFunction(new double[] { 15, 10 }, 7);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 0 }, Relationship.LEQ, 2));
constraints.add(new LinearConstraint(new double[] { 0, 1 }, Relationship.LEQ, 3));
constraints.add(new LinearConstraint(new double[] { 1, 1 }, Relationship.EQ, 4));
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, false);
Assert.assertEquals(2.0, solution.getPoint()[0], 0.0);
Assert.assertEquals(2.0, solution.getPoint()[1], 0.0);
Assert.assertEquals(57.0, solution.getValue(), 0.0);
}
@Test
public void testSingleVariableAndConstraint() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 3 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1 }, Relationship.LEQ, 10));
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, false);
Assert.assertEquals(10.0, solution.getPoint()[0], 0.0);
Assert.assertEquals(30.0, solution.getValue(), 0.0);
}
/**
* With no artificial variables needed (no equals and no greater than
* constraints) we can go straight to Phase 2.
*/
@Test
public void testModelWithNoArtificialVars() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 15, 10 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 0 }, Relationship.LEQ, 2));
constraints.add(new LinearConstraint(new double[] { 0, 1 }, Relationship.LEQ, 3));
constraints.add(new LinearConstraint(new double[] { 1, 1 }, Relationship.LEQ, 4));
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, false);
Assert.assertEquals(2.0, solution.getPoint()[0], 0.0);
Assert.assertEquals(2.0, solution.getPoint()[1], 0.0);
Assert.assertEquals(50.0, solution.getValue(), 0.0);
}
@Test
public void testMinimization() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { -2, 1 }, -5);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 2 }, Relationship.LEQ, 6));
constraints.add(new LinearConstraint(new double[] { 3, 2 }, Relationship.LEQ, 12));
constraints.add(new LinearConstraint(new double[] { 0, 1 }, Relationship.GEQ, 0));
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MINIMIZE, false);
Assert.assertEquals(4.0, solution.getPoint()[0], 0.0);
Assert.assertEquals(0.0, solution.getPoint()[1], 0.0);
Assert.assertEquals(-13.0, solution.getValue(), 0.0);
}
@Test
public void testSolutionWithNegativeDecisionVariable() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { -2, 1 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 1 }, Relationship.GEQ, 6));
constraints.add(new LinearConstraint(new double[] { 1, 2 }, Relationship.LEQ, 14));
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, false);
Assert.assertEquals(-2.0, solution.getPoint()[0], 0.0);
Assert.assertEquals(8.0, solution.getPoint()[1], 0.0);
Assert.assertEquals(12.0, solution.getValue(), 0.0);
}
@Test(expected = NoFeasibleSolutionException.class)
public void testInfeasibleSolution() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 15 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1 }, Relationship.LEQ, 1));
constraints.add(new LinearConstraint(new double[] { 1 }, Relationship.GEQ, 3));
SimplexSolver solver = new SimplexSolver();
solver.optimize(f, constraints, GoalType.MAXIMIZE, false);
}
@Test(expected = UnboundedSolutionException.class)
public void testUnboundedSolution() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 15, 10 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 0 }, Relationship.EQ, 2));
SimplexSolver solver = new SimplexSolver();
solver.optimize(f, constraints, GoalType.MAXIMIZE, false);
}
@Test
public void testRestrictVariablesToNonNegative() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 409, 523, 70, 204, 339 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 43, 56, 345, 56, 5 }, Relationship.LEQ, 4567456));
constraints.add(new LinearConstraint(new double[] { 12, 45, 7, 56, 23 }, Relationship.LEQ, 56454));
constraints.add(new LinearConstraint(new double[] { 8, 768, 0, 34, 7456 }, Relationship.LEQ, 1923421));
constraints.add(new LinearConstraint(new double[] { 12342, 2342, 34, 678, 2342 }, Relationship.GEQ, 4356));
constraints.add(new LinearConstraint(new double[] { 45, 678, 76, 52, 23 }, Relationship.EQ, 456356));
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, true);
Assert.assertEquals(2902.92783505155, solution.getPoint()[0], .0000001);
Assert.assertEquals(480.419243986254, solution.getPoint()[1], .0000001);
Assert.assertEquals(0.0, solution.getPoint()[2], .0000001);
Assert.assertEquals(0.0, solution.getPoint()[3], .0000001);
Assert.assertEquals(0.0, solution.getPoint()[4], .0000001);
Assert.assertEquals(1438556.7491409, solution.getValue(), .0000001);
}
@Test
public void testEpsilon() {
LinearObjectiveFunction f =
new LinearObjectiveFunction(new double[] { 10, 5, 1 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 9, 8, 0 }, Relationship.EQ, 17));
constraints.add(new LinearConstraint(new double[] { 0, 7, 8 }, Relationship.LEQ, 7));
constraints.add(new LinearConstraint(new double[] { 10, 0, 2 }, Relationship.LEQ, 10));
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, false);
Assert.assertEquals(1.0, solution.getPoint()[0], 0.0);
Assert.assertEquals(1.0, solution.getPoint()[1], 0.0);
Assert.assertEquals(0.0, solution.getPoint()[2], 0.0);
Assert.assertEquals(15.0, solution.getValue(), 0.0);
}
@Test
public void testTrivialModel() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 1, 1 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 1 }, Relationship.EQ, 0));
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, true);
Assert.assertEquals(0, solution.getValue(), .0000001);
}
@Test
public void testLargeModel() {
double[] objective = new double[] {
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 12, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
12, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 12, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 12, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 12, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 12, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1};
LinearObjectiveFunction f = new LinearObjectiveFunction(objective, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(equationFromString(objective.length, "x0 + x1 + x2 + x3 - x12 = 0"));
constraints.add(equationFromString(objective.length, "x4 + x5 + x6 + x7 + x8 + x9 + x10 + x11 - x13 = 0"));
constraints.add(equationFromString(objective.length, "x4 + x5 + x6 + x7 + x8 + x9 + x10 + x11 >= 49"));
constraints.add(equationFromString(objective.length, "x0 + x1 + x2 + x3 >= 42"));
constraints.add(equationFromString(objective.length, "x14 + x15 + x16 + x17 - x26 = 0"));
constraints.add(equationFromString(objective.length, "x18 + x19 + x20 + x21 + x22 + x23 + x24 + x25 - x27 = 0"));
constraints.add(equationFromString(objective.length, "x14 + x15 + x16 + x17 - x12 = 0"));
constraints.add(equationFromString(objective.length, "x18 + x19 + x20 + x21 + x22 + x23 + x24 + x25 - x13 = 0"));
constraints.add(equationFromString(objective.length, "x28 + x29 + x30 + x31 - x40 = 0"));
constraints.add(equationFromString(objective.length, "x32 + x33 + x34 + x35 + x36 + x37 + x38 + x39 - x41 = 0"));
constraints.add(equationFromString(objective.length, "x32 + x33 + x34 + x35 + x36 + x37 + x38 + x39 >= 49"));
constraints.add(equationFromString(objective.length, "x28 + x29 + x30 + x31 >= 42"));
constraints.add(equationFromString(objective.length, "x42 + x43 + x44 + x45 - x54 = 0"));
constraints.add(equationFromString(objective.length, "x46 + x47 + x48 + x49 + x50 + x51 + x52 + x53 - x55 = 0"));
constraints.add(equationFromString(objective.length, "x42 + x43 + x44 + x45 - x40 = 0"));
constraints.add(equationFromString(objective.length, "x46 + x47 + x48 + x49 + x50 + x51 + x52 + x53 - x41 = 0"));
constraints.add(equationFromString(objective.length, "x56 + x57 + x58 + x59 - x68 = 0"));
constraints.add(equationFromString(objective.length, "x60 + x61 + x62 + x63 + x64 + x65 + x66 + x67 - x69 = 0"));
constraints.add(equationFromString(objective.length, "x60 + x61 + x62 + x63 + x64 + x65 + x66 + x67 >= 51"));
constraints.add(equationFromString(objective.length, "x56 + x57 + x58 + x59 >= 44"));
constraints.add(equationFromString(objective.length, "x70 + x71 + x72 + x73 - x82 = 0"));
constraints.add(equationFromString(objective.length, "x74 + x75 + x76 + x77 + x78 + x79 + x80 + x81 - x83 = 0"));
constraints.add(equationFromString(objective.length, "x70 + x71 + x72 + x73 - x68 = 0"));
constraints.add(equationFromString(objective.length, "x74 + x75 + x76 + x77 + x78 + x79 + x80 + x81 - x69 = 0"));
constraints.add(equationFromString(objective.length, "x84 + x85 + x86 + x87 - x96 = 0"));
constraints.add(equationFromString(objective.length, "x88 + x89 + x90 + x91 + x92 + x93 + x94 + x95 - x97 = 0"));
constraints.add(equationFromString(objective.length, "x88 + x89 + x90 + x91 + x92 + x93 + x94 + x95 >= 51"));
constraints.add(equationFromString(objective.length, "x84 + x85 + x86 + x87 >= 44"));
constraints.add(equationFromString(objective.length, "x98 + x99 + x100 + x101 - x110 = 0"));
constraints.add(equationFromString(objective.length, "x102 + x103 + x104 + x105 + x106 + x107 + x108 + x109 - x111 = 0"));
constraints.add(equationFromString(objective.length, "x98 + x99 + x100 + x101 - x96 = 0"));
constraints.add(equationFromString(objective.length, "x102 + x103 + x104 + x105 + x106 + x107 + x108 + x109 - x97 = 0"));
constraints.add(equationFromString(objective.length, "x112 + x113 + x114 + x115 - x124 = 0"));
constraints.add(equationFromString(objective.length, "x116 + x117 + x118 + x119 + x120 + x121 + x122 + x123 - x125 = 0"));
constraints.add(equationFromString(objective.length, "x116 + x117 + x118 + x119 + x120 + x121 + x122 + x123 >= 49"));
constraints.add(equationFromString(objective.length, "x112 + x113 + x114 + x115 >= 42"));
constraints.add(equationFromString(objective.length, "x126 + x127 + x128 + x129 - x138 = 0"));
constraints.add(equationFromString(objective.length, "x130 + x131 + x132 + x133 + x134 + x135 + x136 + x137 - x139 = 0"));
constraints.add(equationFromString(objective.length, "x126 + x127 + x128 + x129 - x124 = 0"));
constraints.add(equationFromString(objective.length, "x130 + x131 + x132 + x133 + x134 + x135 + x136 + x137 - x125 = 0"));
constraints.add(equationFromString(objective.length, "x140 + x141 + x142 + x143 - x152 = 0"));
constraints.add(equationFromString(objective.length, "x144 + x145 + x146 + x147 + x148 + x149 + x150 + x151 - x153 = 0"));
constraints.add(equationFromString(objective.length, "x144 + x145 + x146 + x147 + x148 + x149 + x150 + x151 >= 59"));
constraints.add(equationFromString(objective.length, "x140 + x141 + x142 + x143 >= 42"));
constraints.add(equationFromString(objective.length, "x154 + x155 + x156 + x157 - x166 = 0"));
constraints.add(equationFromString(objective.length, "x158 + x159 + x160 + x161 + x162 + x163 + x164 + x165 - x167 = 0"));
constraints.add(equationFromString(objective.length, "x154 + x155 + x156 + x157 - x152 = 0"));
constraints.add(equationFromString(objective.length, "x158 + x159 + x160 + x161 + x162 + x163 + x164 + x165 - x153 = 0"));
constraints.add(equationFromString(objective.length, "x83 + x82 - x168 = 0"));
constraints.add(equationFromString(objective.length, "x111 + x110 - x169 = 0"));
constraints.add(equationFromString(objective.length, "x170 - x182 = 0"));
constraints.add(equationFromString(objective.length, "x171 - x183 = 0"));
constraints.add(equationFromString(objective.length, "x172 - x184 = 0"));
constraints.add(equationFromString(objective.length, "x173 - x185 = 0"));
constraints.add(equationFromString(objective.length, "x174 - x186 = 0"));
constraints.add(equationFromString(objective.length, "x175 + x176 - x187 = 0"));
constraints.add(equationFromString(objective.length, "x177 - x188 = 0"));
constraints.add(equationFromString(objective.length, "x178 - x189 = 0"));
constraints.add(equationFromString(objective.length, "x179 - x190 = 0"));
constraints.add(equationFromString(objective.length, "x180 - x191 = 0"));
constraints.add(equationFromString(objective.length, "x181 - x192 = 0"));
constraints.add(equationFromString(objective.length, "x170 - x26 = 0"));
constraints.add(equationFromString(objective.length, "x171 - x27 = 0"));
constraints.add(equationFromString(objective.length, "x172 - x54 = 0"));
constraints.add(equationFromString(objective.length, "x173 - x55 = 0"));
constraints.add(equationFromString(objective.length, "x174 - x168 = 0"));
constraints.add(equationFromString(objective.length, "x177 - x169 = 0"));
constraints.add(equationFromString(objective.length, "x178 - x138 = 0"));
constraints.add(equationFromString(objective.length, "x179 - x139 = 0"));
constraints.add(equationFromString(objective.length, "x180 - x166 = 0"));
constraints.add(equationFromString(objective.length, "x181 - x167 = 0"));
constraints.add(equationFromString(objective.length, "x193 - x205 = 0"));
constraints.add(equationFromString(objective.length, "x194 - x206 = 0"));
constraints.add(equationFromString(objective.length, "x195 - x207 = 0"));
constraints.add(equationFromString(objective.length, "x196 - x208 = 0"));
constraints.add(equationFromString(objective.length, "x197 - x209 = 0"));
constraints.add(equationFromString(objective.length, "x198 + x199 - x210 = 0"));
constraints.add(equationFromString(objective.length, "x200 - x211 = 0"));
constraints.add(equationFromString(objective.length, "x201 - x212 = 0"));
constraints.add(equationFromString(objective.length, "x202 - x213 = 0"));
constraints.add(equationFromString(objective.length, "x203 - x214 = 0"));
constraints.add(equationFromString(objective.length, "x204 - x215 = 0"));
constraints.add(equationFromString(objective.length, "x193 - x182 = 0"));
constraints.add(equationFromString(objective.length, "x194 - x183 = 0"));
constraints.add(equationFromString(objective.length, "x195 - x184 = 0"));
constraints.add(equationFromString(objective.length, "x196 - x185 = 0"));
constraints.add(equationFromString(objective.length, "x197 - x186 = 0"));
constraints.add(equationFromString(objective.length, "x198 + x199 - x187 = 0"));
constraints.add(equationFromString(objective.length, "x200 - x188 = 0"));
constraints.add(equationFromString(objective.length, "x201 - x189 = 0"));
constraints.add(equationFromString(objective.length, "x202 - x190 = 0"));
constraints.add(equationFromString(objective.length, "x203 - x191 = 0"));
constraints.add(equationFromString(objective.length, "x204 - x192 = 0"));
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MINIMIZE, true);
Assert.assertEquals(7518.0, solution.getValue(), .0000001);
}
/**
* Converts a test string to a {@link LinearConstraint}.
* Ex: x0 + x1 + x2 + x3 - x12 = 0
*/
private LinearConstraint equationFromString(int numCoefficients, String s) {
Relationship relationship;
if (s.contains(">=")) {
relationship = Relationship.GEQ;
} else if (s.contains("<=")) {
relationship = Relationship.LEQ;
} else if (s.contains("=")) {
relationship = Relationship.EQ;
} else {
throw new IllegalArgumentException();
}
String[] equationParts = s.split("[>|<]?=");
double rhs = Double.parseDouble(equationParts[1].trim());
double[] lhs = new double[numCoefficients];
String left = equationParts[0].replaceAll(" ?x", "");
String[] coefficients = left.split(" ");
for (String coefficient : coefficients) {
double value = coefficient.charAt(0) == '-' ? -1 : 1;
int index = Integer.parseInt(coefficient.replaceFirst("[+|-]", "").trim());
lhs[index] = value;
}
return new LinearConstraint(lhs, relationship, rhs);
}
} | // You are a professional Java test case writer, please create a test case named `testMath713NegativeVariable` for the issue `Math-MATH-713`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Math-MATH-713
//
// ## Issue-Title:
// Negative value with restrictNonNegative
//
// ## Issue-Description:
//
// Problem: commons-math-2.2 SimplexSolver.
//
//
// A variable with 0 coefficient may be assigned a negative value nevertheless restrictToNonnegative flag in call:
//
// SimplexSolver.optimize(function, constraints, GoalType.MINIMIZE, true);
//
//
// Function
//
// 1 \* x + 1 \* y + 0
//
//
// Constraints:
//
// 1 \* x + 0 \* y = 1
//
//
// Result:
//
// x = 1; y = -1;
//
//
// Probably variables with 0 coefficients are omitted at some point of computation and because of that the restrictions do not affect their values.
//
//
//
//
//
@Test
public void testMath713NegativeVariable() {
| 43 | 42 | 31 | src/test/java/org/apache/commons/math/optimization/linear/SimplexSolverTest.java | src/test/java | ```markdown
## Issue-ID: Math-MATH-713
## Issue-Title:
Negative value with restrictNonNegative
## Issue-Description:
Problem: commons-math-2.2 SimplexSolver.
A variable with 0 coefficient may be assigned a negative value nevertheless restrictToNonnegative flag in call:
SimplexSolver.optimize(function, constraints, GoalType.MINIMIZE, true);
Function
1 \* x + 1 \* y + 0
Constraints:
1 \* x + 0 \* y = 1
Result:
x = 1; y = -1;
Probably variables with 0 coefficients are omitted at some point of computation and because of that the restrictions do not affect their values.
```
You are a professional Java test case writer, please create a test case named `testMath713NegativeVariable` for the issue `Math-MATH-713`, utilizing the provided issue report information and the following function signature.
```java
@Test
public void testMath713NegativeVariable() {
```
| 31 | [
"org.apache.commons.math.optimization.linear.SimplexTableau"
] | 9c5f6389544e0fd6a8f62967207becb69c2379f51c4f9924c6a093630312e0da | @Test
public void testMath713NegativeVariable() | // You are a professional Java test case writer, please create a test case named `testMath713NegativeVariable` for the issue `Math-MATH-713`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Math-MATH-713
//
// ## Issue-Title:
// Negative value with restrictNonNegative
//
// ## Issue-Description:
//
// Problem: commons-math-2.2 SimplexSolver.
//
//
// A variable with 0 coefficient may be assigned a negative value nevertheless restrictToNonnegative flag in call:
//
// SimplexSolver.optimize(function, constraints, GoalType.MINIMIZE, true);
//
//
// Function
//
// 1 \* x + 1 \* y + 0
//
//
// Constraints:
//
// 1 \* x + 0 \* y = 1
//
//
// Result:
//
// x = 1; y = -1;
//
//
// Probably variables with 0 coefficients are omitted at some point of computation and because of that the restrictions do not affect their values.
//
//
//
//
//
| Math | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.math.optimization.linear;
import org.junit.Assert;
import java.util.ArrayList;
import java.util.Collection;
import org.apache.commons.math.optimization.GoalType;
import org.apache.commons.math.optimization.RealPointValuePair;
import org.apache.commons.math.util.Precision;
import org.junit.Test;
public class SimplexSolverTest {
@Test
public void testMath713NegativeVariable() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] {1.0, 1.0}, 0.0d);
ArrayList<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] {1, 0}, Relationship.EQ, 1));
double epsilon = 1e-6;
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MINIMIZE, true);
Assert.assertTrue(Precision.compareTo(solution.getPoint()[0], 0.0d, epsilon) >= 0);
Assert.assertTrue(Precision.compareTo(solution.getPoint()[1], 0.0d, epsilon) >= 0);
}
@Test
public void testMath434NegativeVariable() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] {0.0, 0.0, 1.0}, 0.0d);
ArrayList<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] {1, 1, 0}, Relationship.EQ, 5));
constraints.add(new LinearConstraint(new double[] {0, 0, 1}, Relationship.GEQ, -10));
double epsilon = 1e-6;
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MINIMIZE, false);
Assert.assertEquals(5.0, solution.getPoint()[0] + solution.getPoint()[1], epsilon);
Assert.assertEquals(-10.0, solution.getPoint()[2], epsilon);
Assert.assertEquals(-10.0, solution.getValue(), epsilon);
}
@Test(expected = NoFeasibleSolutionException.class)
public void testMath434UnfeasibleSolution() {
double epsilon = 1e-6;
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] {1.0, 0.0}, 0.0);
ArrayList<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] {epsilon/2, 0.5}, Relationship.EQ, 0));
constraints.add(new LinearConstraint(new double[] {1e-3, 0.1}, Relationship.EQ, 10));
SimplexSolver solver = new SimplexSolver();
// allowing only non-negative values, no feasible solution shall be found
solver.optimize(f, constraints, GoalType.MINIMIZE, true);
}
@Test
public void testMath434PivotRowSelection() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] {1.0}, 0.0);
double epsilon = 1e-6;
ArrayList<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] {200}, Relationship.GEQ, 1));
constraints.add(new LinearConstraint(new double[] {100}, Relationship.GEQ, 0.499900001));
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MINIMIZE, false);
Assert.assertTrue(Precision.compareTo(solution.getPoint()[0] * 200.d, 1.d, epsilon) >= 0);
Assert.assertEquals(0.0050, solution.getValue(), epsilon);
}
@Test
public void testMath434PivotRowSelection2() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] {0.0d, 1.0d, 1.0d, 0.0d, 0.0d, 0.0d, 0.0d}, 0.0d);
ArrayList<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] {1.0d, -0.1d, 0.0d, 0.0d, 0.0d, 0.0d, 0.0d}, Relationship.EQ, -0.1d));
constraints.add(new LinearConstraint(new double[] {1.0d, 0.0d, 0.0d, 0.0d, 0.0d, 0.0d, 0.0d}, Relationship.GEQ, -1e-18d));
constraints.add(new LinearConstraint(new double[] {0.0d, 1.0d, 0.0d, 0.0d, 0.0d, 0.0d, 0.0d}, Relationship.GEQ, 0.0d));
constraints.add(new LinearConstraint(new double[] {0.0d, 0.0d, 0.0d, 1.0d, 0.0d, -0.0128588d, 1e-5d}, Relationship.EQ, 0.0d));
constraints.add(new LinearConstraint(new double[] {0.0d, 0.0d, 0.0d, 0.0d, 1.0d, 1e-5d, -0.0128586d}, Relationship.EQ, 1e-10d));
constraints.add(new LinearConstraint(new double[] {0.0d, 0.0d, 1.0d, -1.0d, 0.0d, 0.0d, 0.0d}, Relationship.GEQ, 0.0d));
constraints.add(new LinearConstraint(new double[] {0.0d, 0.0d, 1.0d, 1.0d, 0.0d, 0.0d, 0.0d}, Relationship.GEQ, 0.0d));
constraints.add(new LinearConstraint(new double[] {0.0d, 0.0d, 1.0d, 0.0d, -1.0d, 0.0d, 0.0d}, Relationship.GEQ, 0.0d));
constraints.add(new LinearConstraint(new double[] {0.0d, 0.0d, 1.0d, 0.0d, 1.0d, 0.0d, 0.0d}, Relationship.GEQ, 0.0d));
double epsilon = 1e-7;
SimplexSolver simplex = new SimplexSolver();
RealPointValuePair solution = simplex.optimize(f, constraints, GoalType.MINIMIZE, false);
Assert.assertTrue(Precision.compareTo(solution.getPoint()[0], -1e-18d, epsilon) >= 0);
Assert.assertEquals(1.0d, solution.getPoint()[1], epsilon);
Assert.assertEquals(0.0d, solution.getPoint()[2], epsilon);
Assert.assertEquals(1.0d, solution.getValue(), epsilon);
}
@Test
public void testMath272() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 2, 2, 1 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 1, 0 }, Relationship.GEQ, 1));
constraints.add(new LinearConstraint(new double[] { 1, 0, 1 }, Relationship.GEQ, 1));
constraints.add(new LinearConstraint(new double[] { 0, 1, 0 }, Relationship.GEQ, 1));
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MINIMIZE, true);
Assert.assertEquals(0.0, solution.getPoint()[0], .0000001);
Assert.assertEquals(1.0, solution.getPoint()[1], .0000001);
Assert.assertEquals(1.0, solution.getPoint()[2], .0000001);
Assert.assertEquals(3.0, solution.getValue(), .0000001);
}
@Test
public void testMath286() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 0.8, 0.2, 0.7, 0.3, 0.6, 0.4 }, 0 );
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 0, 1, 0, 1, 0 }, Relationship.EQ, 23.0));
constraints.add(new LinearConstraint(new double[] { 0, 1, 0, 1, 0, 1 }, Relationship.EQ, 23.0));
constraints.add(new LinearConstraint(new double[] { 1, 0, 0, 0, 0, 0 }, Relationship.GEQ, 10.0));
constraints.add(new LinearConstraint(new double[] { 0, 0, 1, 0, 0, 0 }, Relationship.GEQ, 8.0));
constraints.add(new LinearConstraint(new double[] { 0, 0, 0, 0, 1, 0 }, Relationship.GEQ, 5.0));
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, true);
Assert.assertEquals(25.8, solution.getValue(), .0000001);
Assert.assertEquals(23.0, solution.getPoint()[0] + solution.getPoint()[2] + solution.getPoint()[4], 0.0000001);
Assert.assertEquals(23.0, solution.getPoint()[1] + solution.getPoint()[3] + solution.getPoint()[5], 0.0000001);
Assert.assertTrue(solution.getPoint()[0] >= 10.0 - 0.0000001);
Assert.assertTrue(solution.getPoint()[2] >= 8.0 - 0.0000001);
Assert.assertTrue(solution.getPoint()[4] >= 5.0 - 0.0000001);
}
@Test
public void testDegeneracy() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 0.8, 0.7 }, 0 );
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 1 }, Relationship.LEQ, 18.0));
constraints.add(new LinearConstraint(new double[] { 1, 0 }, Relationship.GEQ, 10.0));
constraints.add(new LinearConstraint(new double[] { 0, 1 }, Relationship.GEQ, 8.0));
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, true);
Assert.assertEquals(13.6, solution.getValue(), .0000001);
}
@Test
public void testMath288() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 7, 3, 0, 0 }, 0 );
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 3, 0, -5, 0 }, Relationship.LEQ, 0.0));
constraints.add(new LinearConstraint(new double[] { 2, 0, 0, -5 }, Relationship.LEQ, 0.0));
constraints.add(new LinearConstraint(new double[] { 0, 3, 0, -5 }, Relationship.LEQ, 0.0));
constraints.add(new LinearConstraint(new double[] { 1, 0, 0, 0 }, Relationship.LEQ, 1.0));
constraints.add(new LinearConstraint(new double[] { 0, 1, 0, 0 }, Relationship.LEQ, 1.0));
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, true);
Assert.assertEquals(10.0, solution.getValue(), .0000001);
}
@Test
public void testMath290GEQ() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 1, 5 }, 0 );
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 2, 0 }, Relationship.GEQ, -1.0));
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MINIMIZE, true);
Assert.assertEquals(0, solution.getValue(), .0000001);
Assert.assertEquals(0, solution.getPoint()[0], .0000001);
Assert.assertEquals(0, solution.getPoint()[1], .0000001);
}
@Test(expected=NoFeasibleSolutionException.class)
public void testMath290LEQ() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 1, 5 }, 0 );
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 2, 0 }, Relationship.LEQ, -1.0));
SimplexSolver solver = new SimplexSolver();
solver.optimize(f, constraints, GoalType.MINIMIZE, true);
}
@Test
public void testMath293() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 0.8, 0.2, 0.7, 0.3, 0.4, 0.6}, 0 );
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 0, 1, 0, 1, 0 }, Relationship.EQ, 30.0));
constraints.add(new LinearConstraint(new double[] { 0, 1, 0, 1, 0, 1 }, Relationship.EQ, 30.0));
constraints.add(new LinearConstraint(new double[] { 0.8, 0.2, 0.0, 0.0, 0.0, 0.0 }, Relationship.GEQ, 10.0));
constraints.add(new LinearConstraint(new double[] { 0.0, 0.0, 0.7, 0.3, 0.0, 0.0 }, Relationship.GEQ, 10.0));
constraints.add(new LinearConstraint(new double[] { 0.0, 0.0, 0.0, 0.0, 0.4, 0.6 }, Relationship.GEQ, 10.0));
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution1 = solver.optimize(f, constraints, GoalType.MAXIMIZE, true);
Assert.assertEquals(15.7143, solution1.getPoint()[0], .0001);
Assert.assertEquals(0.0, solution1.getPoint()[1], .0001);
Assert.assertEquals(14.2857, solution1.getPoint()[2], .0001);
Assert.assertEquals(0.0, solution1.getPoint()[3], .0001);
Assert.assertEquals(0.0, solution1.getPoint()[4], .0001);
Assert.assertEquals(30.0, solution1.getPoint()[5], .0001);
Assert.assertEquals(40.57143, solution1.getValue(), .0001);
double valA = 0.8 * solution1.getPoint()[0] + 0.2 * solution1.getPoint()[1];
double valB = 0.7 * solution1.getPoint()[2] + 0.3 * solution1.getPoint()[3];
double valC = 0.4 * solution1.getPoint()[4] + 0.6 * solution1.getPoint()[5];
f = new LinearObjectiveFunction(new double[] { 0.8, 0.2, 0.7, 0.3, 0.4, 0.6}, 0 );
constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 0, 1, 0, 1, 0 }, Relationship.EQ, 30.0));
constraints.add(new LinearConstraint(new double[] { 0, 1, 0, 1, 0, 1 }, Relationship.EQ, 30.0));
constraints.add(new LinearConstraint(new double[] { 0.8, 0.2, 0.0, 0.0, 0.0, 0.0 }, Relationship.GEQ, valA));
constraints.add(new LinearConstraint(new double[] { 0.0, 0.0, 0.7, 0.3, 0.0, 0.0 }, Relationship.GEQ, valB));
constraints.add(new LinearConstraint(new double[] { 0.0, 0.0, 0.0, 0.0, 0.4, 0.6 }, Relationship.GEQ, valC));
RealPointValuePair solution2 = solver.optimize(f, constraints, GoalType.MAXIMIZE, true);
Assert.assertEquals(40.57143, solution2.getValue(), .0001);
}
@Test
public void testSimplexSolver() {
LinearObjectiveFunction f =
new LinearObjectiveFunction(new double[] { 15, 10 }, 7);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 0 }, Relationship.LEQ, 2));
constraints.add(new LinearConstraint(new double[] { 0, 1 }, Relationship.LEQ, 3));
constraints.add(new LinearConstraint(new double[] { 1, 1 }, Relationship.EQ, 4));
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, false);
Assert.assertEquals(2.0, solution.getPoint()[0], 0.0);
Assert.assertEquals(2.0, solution.getPoint()[1], 0.0);
Assert.assertEquals(57.0, solution.getValue(), 0.0);
}
@Test
public void testSingleVariableAndConstraint() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 3 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1 }, Relationship.LEQ, 10));
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, false);
Assert.assertEquals(10.0, solution.getPoint()[0], 0.0);
Assert.assertEquals(30.0, solution.getValue(), 0.0);
}
/**
* With no artificial variables needed (no equals and no greater than
* constraints) we can go straight to Phase 2.
*/
@Test
public void testModelWithNoArtificialVars() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 15, 10 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 0 }, Relationship.LEQ, 2));
constraints.add(new LinearConstraint(new double[] { 0, 1 }, Relationship.LEQ, 3));
constraints.add(new LinearConstraint(new double[] { 1, 1 }, Relationship.LEQ, 4));
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, false);
Assert.assertEquals(2.0, solution.getPoint()[0], 0.0);
Assert.assertEquals(2.0, solution.getPoint()[1], 0.0);
Assert.assertEquals(50.0, solution.getValue(), 0.0);
}
@Test
public void testMinimization() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { -2, 1 }, -5);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 2 }, Relationship.LEQ, 6));
constraints.add(new LinearConstraint(new double[] { 3, 2 }, Relationship.LEQ, 12));
constraints.add(new LinearConstraint(new double[] { 0, 1 }, Relationship.GEQ, 0));
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MINIMIZE, false);
Assert.assertEquals(4.0, solution.getPoint()[0], 0.0);
Assert.assertEquals(0.0, solution.getPoint()[1], 0.0);
Assert.assertEquals(-13.0, solution.getValue(), 0.0);
}
@Test
public void testSolutionWithNegativeDecisionVariable() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { -2, 1 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 1 }, Relationship.GEQ, 6));
constraints.add(new LinearConstraint(new double[] { 1, 2 }, Relationship.LEQ, 14));
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, false);
Assert.assertEquals(-2.0, solution.getPoint()[0], 0.0);
Assert.assertEquals(8.0, solution.getPoint()[1], 0.0);
Assert.assertEquals(12.0, solution.getValue(), 0.0);
}
@Test(expected = NoFeasibleSolutionException.class)
public void testInfeasibleSolution() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 15 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1 }, Relationship.LEQ, 1));
constraints.add(new LinearConstraint(new double[] { 1 }, Relationship.GEQ, 3));
SimplexSolver solver = new SimplexSolver();
solver.optimize(f, constraints, GoalType.MAXIMIZE, false);
}
@Test(expected = UnboundedSolutionException.class)
public void testUnboundedSolution() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 15, 10 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 0 }, Relationship.EQ, 2));
SimplexSolver solver = new SimplexSolver();
solver.optimize(f, constraints, GoalType.MAXIMIZE, false);
}
@Test
public void testRestrictVariablesToNonNegative() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 409, 523, 70, 204, 339 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 43, 56, 345, 56, 5 }, Relationship.LEQ, 4567456));
constraints.add(new LinearConstraint(new double[] { 12, 45, 7, 56, 23 }, Relationship.LEQ, 56454));
constraints.add(new LinearConstraint(new double[] { 8, 768, 0, 34, 7456 }, Relationship.LEQ, 1923421));
constraints.add(new LinearConstraint(new double[] { 12342, 2342, 34, 678, 2342 }, Relationship.GEQ, 4356));
constraints.add(new LinearConstraint(new double[] { 45, 678, 76, 52, 23 }, Relationship.EQ, 456356));
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, true);
Assert.assertEquals(2902.92783505155, solution.getPoint()[0], .0000001);
Assert.assertEquals(480.419243986254, solution.getPoint()[1], .0000001);
Assert.assertEquals(0.0, solution.getPoint()[2], .0000001);
Assert.assertEquals(0.0, solution.getPoint()[3], .0000001);
Assert.assertEquals(0.0, solution.getPoint()[4], .0000001);
Assert.assertEquals(1438556.7491409, solution.getValue(), .0000001);
}
@Test
public void testEpsilon() {
LinearObjectiveFunction f =
new LinearObjectiveFunction(new double[] { 10, 5, 1 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 9, 8, 0 }, Relationship.EQ, 17));
constraints.add(new LinearConstraint(new double[] { 0, 7, 8 }, Relationship.LEQ, 7));
constraints.add(new LinearConstraint(new double[] { 10, 0, 2 }, Relationship.LEQ, 10));
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, false);
Assert.assertEquals(1.0, solution.getPoint()[0], 0.0);
Assert.assertEquals(1.0, solution.getPoint()[1], 0.0);
Assert.assertEquals(0.0, solution.getPoint()[2], 0.0);
Assert.assertEquals(15.0, solution.getValue(), 0.0);
}
@Test
public void testTrivialModel() {
LinearObjectiveFunction f = new LinearObjectiveFunction(new double[] { 1, 1 }, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(new LinearConstraint(new double[] { 1, 1 }, Relationship.EQ, 0));
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MAXIMIZE, true);
Assert.assertEquals(0, solution.getValue(), .0000001);
}
@Test
public void testLargeModel() {
double[] objective = new double[] {
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 12, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
12, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 12, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 12, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 12, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 12, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1};
LinearObjectiveFunction f = new LinearObjectiveFunction(objective, 0);
Collection<LinearConstraint> constraints = new ArrayList<LinearConstraint>();
constraints.add(equationFromString(objective.length, "x0 + x1 + x2 + x3 - x12 = 0"));
constraints.add(equationFromString(objective.length, "x4 + x5 + x6 + x7 + x8 + x9 + x10 + x11 - x13 = 0"));
constraints.add(equationFromString(objective.length, "x4 + x5 + x6 + x7 + x8 + x9 + x10 + x11 >= 49"));
constraints.add(equationFromString(objective.length, "x0 + x1 + x2 + x3 >= 42"));
constraints.add(equationFromString(objective.length, "x14 + x15 + x16 + x17 - x26 = 0"));
constraints.add(equationFromString(objective.length, "x18 + x19 + x20 + x21 + x22 + x23 + x24 + x25 - x27 = 0"));
constraints.add(equationFromString(objective.length, "x14 + x15 + x16 + x17 - x12 = 0"));
constraints.add(equationFromString(objective.length, "x18 + x19 + x20 + x21 + x22 + x23 + x24 + x25 - x13 = 0"));
constraints.add(equationFromString(objective.length, "x28 + x29 + x30 + x31 - x40 = 0"));
constraints.add(equationFromString(objective.length, "x32 + x33 + x34 + x35 + x36 + x37 + x38 + x39 - x41 = 0"));
constraints.add(equationFromString(objective.length, "x32 + x33 + x34 + x35 + x36 + x37 + x38 + x39 >= 49"));
constraints.add(equationFromString(objective.length, "x28 + x29 + x30 + x31 >= 42"));
constraints.add(equationFromString(objective.length, "x42 + x43 + x44 + x45 - x54 = 0"));
constraints.add(equationFromString(objective.length, "x46 + x47 + x48 + x49 + x50 + x51 + x52 + x53 - x55 = 0"));
constraints.add(equationFromString(objective.length, "x42 + x43 + x44 + x45 - x40 = 0"));
constraints.add(equationFromString(objective.length, "x46 + x47 + x48 + x49 + x50 + x51 + x52 + x53 - x41 = 0"));
constraints.add(equationFromString(objective.length, "x56 + x57 + x58 + x59 - x68 = 0"));
constraints.add(equationFromString(objective.length, "x60 + x61 + x62 + x63 + x64 + x65 + x66 + x67 - x69 = 0"));
constraints.add(equationFromString(objective.length, "x60 + x61 + x62 + x63 + x64 + x65 + x66 + x67 >= 51"));
constraints.add(equationFromString(objective.length, "x56 + x57 + x58 + x59 >= 44"));
constraints.add(equationFromString(objective.length, "x70 + x71 + x72 + x73 - x82 = 0"));
constraints.add(equationFromString(objective.length, "x74 + x75 + x76 + x77 + x78 + x79 + x80 + x81 - x83 = 0"));
constraints.add(equationFromString(objective.length, "x70 + x71 + x72 + x73 - x68 = 0"));
constraints.add(equationFromString(objective.length, "x74 + x75 + x76 + x77 + x78 + x79 + x80 + x81 - x69 = 0"));
constraints.add(equationFromString(objective.length, "x84 + x85 + x86 + x87 - x96 = 0"));
constraints.add(equationFromString(objective.length, "x88 + x89 + x90 + x91 + x92 + x93 + x94 + x95 - x97 = 0"));
constraints.add(equationFromString(objective.length, "x88 + x89 + x90 + x91 + x92 + x93 + x94 + x95 >= 51"));
constraints.add(equationFromString(objective.length, "x84 + x85 + x86 + x87 >= 44"));
constraints.add(equationFromString(objective.length, "x98 + x99 + x100 + x101 - x110 = 0"));
constraints.add(equationFromString(objective.length, "x102 + x103 + x104 + x105 + x106 + x107 + x108 + x109 - x111 = 0"));
constraints.add(equationFromString(objective.length, "x98 + x99 + x100 + x101 - x96 = 0"));
constraints.add(equationFromString(objective.length, "x102 + x103 + x104 + x105 + x106 + x107 + x108 + x109 - x97 = 0"));
constraints.add(equationFromString(objective.length, "x112 + x113 + x114 + x115 - x124 = 0"));
constraints.add(equationFromString(objective.length, "x116 + x117 + x118 + x119 + x120 + x121 + x122 + x123 - x125 = 0"));
constraints.add(equationFromString(objective.length, "x116 + x117 + x118 + x119 + x120 + x121 + x122 + x123 >= 49"));
constraints.add(equationFromString(objective.length, "x112 + x113 + x114 + x115 >= 42"));
constraints.add(equationFromString(objective.length, "x126 + x127 + x128 + x129 - x138 = 0"));
constraints.add(equationFromString(objective.length, "x130 + x131 + x132 + x133 + x134 + x135 + x136 + x137 - x139 = 0"));
constraints.add(equationFromString(objective.length, "x126 + x127 + x128 + x129 - x124 = 0"));
constraints.add(equationFromString(objective.length, "x130 + x131 + x132 + x133 + x134 + x135 + x136 + x137 - x125 = 0"));
constraints.add(equationFromString(objective.length, "x140 + x141 + x142 + x143 - x152 = 0"));
constraints.add(equationFromString(objective.length, "x144 + x145 + x146 + x147 + x148 + x149 + x150 + x151 - x153 = 0"));
constraints.add(equationFromString(objective.length, "x144 + x145 + x146 + x147 + x148 + x149 + x150 + x151 >= 59"));
constraints.add(equationFromString(objective.length, "x140 + x141 + x142 + x143 >= 42"));
constraints.add(equationFromString(objective.length, "x154 + x155 + x156 + x157 - x166 = 0"));
constraints.add(equationFromString(objective.length, "x158 + x159 + x160 + x161 + x162 + x163 + x164 + x165 - x167 = 0"));
constraints.add(equationFromString(objective.length, "x154 + x155 + x156 + x157 - x152 = 0"));
constraints.add(equationFromString(objective.length, "x158 + x159 + x160 + x161 + x162 + x163 + x164 + x165 - x153 = 0"));
constraints.add(equationFromString(objective.length, "x83 + x82 - x168 = 0"));
constraints.add(equationFromString(objective.length, "x111 + x110 - x169 = 0"));
constraints.add(equationFromString(objective.length, "x170 - x182 = 0"));
constraints.add(equationFromString(objective.length, "x171 - x183 = 0"));
constraints.add(equationFromString(objective.length, "x172 - x184 = 0"));
constraints.add(equationFromString(objective.length, "x173 - x185 = 0"));
constraints.add(equationFromString(objective.length, "x174 - x186 = 0"));
constraints.add(equationFromString(objective.length, "x175 + x176 - x187 = 0"));
constraints.add(equationFromString(objective.length, "x177 - x188 = 0"));
constraints.add(equationFromString(objective.length, "x178 - x189 = 0"));
constraints.add(equationFromString(objective.length, "x179 - x190 = 0"));
constraints.add(equationFromString(objective.length, "x180 - x191 = 0"));
constraints.add(equationFromString(objective.length, "x181 - x192 = 0"));
constraints.add(equationFromString(objective.length, "x170 - x26 = 0"));
constraints.add(equationFromString(objective.length, "x171 - x27 = 0"));
constraints.add(equationFromString(objective.length, "x172 - x54 = 0"));
constraints.add(equationFromString(objective.length, "x173 - x55 = 0"));
constraints.add(equationFromString(objective.length, "x174 - x168 = 0"));
constraints.add(equationFromString(objective.length, "x177 - x169 = 0"));
constraints.add(equationFromString(objective.length, "x178 - x138 = 0"));
constraints.add(equationFromString(objective.length, "x179 - x139 = 0"));
constraints.add(equationFromString(objective.length, "x180 - x166 = 0"));
constraints.add(equationFromString(objective.length, "x181 - x167 = 0"));
constraints.add(equationFromString(objective.length, "x193 - x205 = 0"));
constraints.add(equationFromString(objective.length, "x194 - x206 = 0"));
constraints.add(equationFromString(objective.length, "x195 - x207 = 0"));
constraints.add(equationFromString(objective.length, "x196 - x208 = 0"));
constraints.add(equationFromString(objective.length, "x197 - x209 = 0"));
constraints.add(equationFromString(objective.length, "x198 + x199 - x210 = 0"));
constraints.add(equationFromString(objective.length, "x200 - x211 = 0"));
constraints.add(equationFromString(objective.length, "x201 - x212 = 0"));
constraints.add(equationFromString(objective.length, "x202 - x213 = 0"));
constraints.add(equationFromString(objective.length, "x203 - x214 = 0"));
constraints.add(equationFromString(objective.length, "x204 - x215 = 0"));
constraints.add(equationFromString(objective.length, "x193 - x182 = 0"));
constraints.add(equationFromString(objective.length, "x194 - x183 = 0"));
constraints.add(equationFromString(objective.length, "x195 - x184 = 0"));
constraints.add(equationFromString(objective.length, "x196 - x185 = 0"));
constraints.add(equationFromString(objective.length, "x197 - x186 = 0"));
constraints.add(equationFromString(objective.length, "x198 + x199 - x187 = 0"));
constraints.add(equationFromString(objective.length, "x200 - x188 = 0"));
constraints.add(equationFromString(objective.length, "x201 - x189 = 0"));
constraints.add(equationFromString(objective.length, "x202 - x190 = 0"));
constraints.add(equationFromString(objective.length, "x203 - x191 = 0"));
constraints.add(equationFromString(objective.length, "x204 - x192 = 0"));
SimplexSolver solver = new SimplexSolver();
RealPointValuePair solution = solver.optimize(f, constraints, GoalType.MINIMIZE, true);
Assert.assertEquals(7518.0, solution.getValue(), .0000001);
}
/**
* Converts a test string to a {@link LinearConstraint}.
* Ex: x0 + x1 + x2 + x3 - x12 = 0
*/
private LinearConstraint equationFromString(int numCoefficients, String s) {
Relationship relationship;
if (s.contains(">=")) {
relationship = Relationship.GEQ;
} else if (s.contains("<=")) {
relationship = Relationship.LEQ;
} else if (s.contains("=")) {
relationship = Relationship.EQ;
} else {
throw new IllegalArgumentException();
}
String[] equationParts = s.split("[>|<]?=");
double rhs = Double.parseDouble(equationParts[1].trim());
double[] lhs = new double[numCoefficients];
String left = equationParts[0].replaceAll(" ?x", "");
String[] coefficients = left.split(" ");
for (String coefficient : coefficients) {
double value = coefficient.charAt(0) == '-' ? -1 : 1;
int index = Integer.parseInt(coefficient.replaceFirst("[+|-]", "").trim());
lhs[index] = value;
}
return new LinearConstraint(lhs, relationship, rhs);
}
} |
||
@Test
public void testEqualsCS1() {
Assert.assertFalse(StringUtils.equals(new StringBuilder("abc"), null));
Assert.assertFalse(StringUtils.equals(null, new StringBuilder("abc")));
Assert.assertTrue(StringUtils.equals(new StringBuilder("abc"), new StringBuilder("abc")));
Assert.assertFalse(StringUtils.equals(new StringBuilder("abc"), new StringBuilder("abcd")));
Assert.assertFalse(StringUtils.equals(new StringBuilder("abcd"), new StringBuilder("abc")));
Assert.assertFalse(StringUtils.equals(new StringBuilder("abc"), new StringBuilder("ABC")));
} | org.apache.commons.codec.binary.StringUtilsTest::testEqualsCS1 | src/test/java/org/apache/commons/codec/binary/StringUtilsTest.java | 230 | src/test/java/org/apache/commons/codec/binary/StringUtilsTest.java | testEqualsCS1 | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.codec.binary;
import java.io.UnsupportedEncodingException;
import java.util.Arrays;
import org.junit.Assert;
import org.junit.Test;
/**
* Tests {@link StringUtils}
*
* @version $Id$
*/
public class StringUtilsTest {
private static final byte[] BYTES_FIXTURE = {'a','b','c'};
// This is valid input for UTF-16BE
private static final byte[] BYTES_FIXTURE_16BE = {0, 'a', 0, 'b', 0, 'c'};
// This is valid for UTF-16LE
private static final byte[] BYTES_FIXTURE_16LE = {'a', 0, 'b', 0, 'c', 0};
private static final String STRING_FIXTURE = "ABC";
/**
* We could make the constructor private but there does not seem to be a point to jumping through extra code hoops
* to restrict instantiation right now.
*/
@Test
public void testConstructor() {
new StringUtils();
}
@Test
public void testGetBytesIso8859_1() throws UnsupportedEncodingException {
final String charsetName = "ISO-8859-1";
testGetBytesUnchecked(charsetName);
final byte[] expected = STRING_FIXTURE.getBytes(charsetName);
final byte[] actual = StringUtils.getBytesIso8859_1(STRING_FIXTURE);
Assert.assertTrue(Arrays.equals(expected, actual));
}
private void testGetBytesUnchecked(final String charsetName) throws UnsupportedEncodingException {
final byte[] expected = STRING_FIXTURE.getBytes(charsetName);
final byte[] actual = StringUtils.getBytesUnchecked(STRING_FIXTURE, charsetName);
Assert.assertTrue(Arrays.equals(expected, actual));
}
@Test
public void testGetBytesUsAscii() throws UnsupportedEncodingException {
final String charsetName = "US-ASCII";
testGetBytesUnchecked(charsetName);
final byte[] expected = STRING_FIXTURE.getBytes(charsetName);
final byte[] actual = StringUtils.getBytesUsAscii(STRING_FIXTURE);
Assert.assertTrue(Arrays.equals(expected, actual));
}
@Test
public void testGetBytesUtf16() throws UnsupportedEncodingException {
final String charsetName = "UTF-16";
testGetBytesUnchecked(charsetName);
final byte[] expected = STRING_FIXTURE.getBytes(charsetName);
final byte[] actual = StringUtils.getBytesUtf16(STRING_FIXTURE);
Assert.assertTrue(Arrays.equals(expected, actual));
}
@Test
public void testGetBytesUtf16Be() throws UnsupportedEncodingException {
final String charsetName = "UTF-16BE";
testGetBytesUnchecked(charsetName);
final byte[] expected = STRING_FIXTURE.getBytes(charsetName);
final byte[] actual = StringUtils.getBytesUtf16Be(STRING_FIXTURE);
Assert.assertTrue(Arrays.equals(expected, actual));
}
@Test
public void testGetBytesUtf16Le() throws UnsupportedEncodingException {
final String charsetName = "UTF-16LE";
testGetBytesUnchecked(charsetName);
final byte[] expected = STRING_FIXTURE.getBytes(charsetName);
final byte[] actual = StringUtils.getBytesUtf16Le(STRING_FIXTURE);
Assert.assertTrue(Arrays.equals(expected, actual));
}
@Test
public void testGetBytesUtf8() throws UnsupportedEncodingException {
final String charsetName = "UTF-8";
testGetBytesUnchecked(charsetName);
final byte[] expected = STRING_FIXTURE.getBytes(charsetName);
final byte[] actual = StringUtils.getBytesUtf8(STRING_FIXTURE);
Assert.assertTrue(Arrays.equals(expected, actual));
}
@Test
public void testGetBytesUncheckedBadName() {
try {
StringUtils.getBytesUnchecked(STRING_FIXTURE, "UNKNOWN");
Assert.fail("Expected " + IllegalStateException.class.getName());
} catch (final IllegalStateException e) {
// Expected
}
}
@Test
public void testGetBytesUncheckedNullInput() {
Assert.assertNull(StringUtils.getBytesUnchecked(null, "UNKNOWN"));
}
private void testNewString(final String charsetName) throws UnsupportedEncodingException {
final String expected = new String(BYTES_FIXTURE, charsetName);
final String actual = StringUtils.newString(BYTES_FIXTURE, charsetName);
Assert.assertEquals(expected, actual);
}
@Test
public void testNewStringBadEnc() {
try {
StringUtils.newString(BYTES_FIXTURE, "UNKNOWN");
Assert.fail("Expected " + IllegalStateException.class.getName());
} catch (final IllegalStateException e) {
// Expected
}
}
@Test
public void testNewStringNullInput() {
Assert.assertNull(StringUtils.newString(null, "UNKNOWN"));
}
@Test
public void testNewStringNullInput_CODEC229() {
Assert.assertNull(StringUtils.newStringUtf8(null));
Assert.assertNull(StringUtils.newStringIso8859_1(null));
Assert.assertNull(StringUtils.newStringUsAscii(null));
Assert.assertNull(StringUtils.newStringUtf16(null));
Assert.assertNull(StringUtils.newStringUtf16Be(null));
Assert.assertNull(StringUtils.newStringUtf16Le(null));
}
@Test
public void testNewStringIso8859_1() throws UnsupportedEncodingException {
final String charsetName = "ISO-8859-1";
testNewString(charsetName);
final String expected = new String(BYTES_FIXTURE, charsetName);
final String actual = StringUtils.newStringIso8859_1(BYTES_FIXTURE);
Assert.assertEquals(expected, actual);
}
@Test
public void testNewStringUsAscii() throws UnsupportedEncodingException {
final String charsetName = "US-ASCII";
testNewString(charsetName);
final String expected = new String(BYTES_FIXTURE, charsetName);
final String actual = StringUtils.newStringUsAscii(BYTES_FIXTURE);
Assert.assertEquals(expected, actual);
}
@Test
public void testNewStringUtf16() throws UnsupportedEncodingException {
final String charsetName = "UTF-16";
testNewString(charsetName);
final String expected = new String(BYTES_FIXTURE, charsetName);
final String actual = StringUtils.newStringUtf16(BYTES_FIXTURE);
Assert.assertEquals(expected, actual);
}
@Test
public void testNewStringUtf16Be() throws UnsupportedEncodingException {
final String charsetName = "UTF-16BE";
testNewString(charsetName);
final String expected = new String(BYTES_FIXTURE_16BE, charsetName);
final String actual = StringUtils.newStringUtf16Be(BYTES_FIXTURE_16BE);
Assert.assertEquals(expected, actual);
}
@Test
public void testNewStringUtf16Le() throws UnsupportedEncodingException {
final String charsetName = "UTF-16LE";
testNewString(charsetName);
final String expected = new String(BYTES_FIXTURE_16LE, charsetName);
final String actual = StringUtils.newStringUtf16Le(BYTES_FIXTURE_16LE);
Assert.assertEquals(expected, actual);
}
@Test
public void testNewStringUtf8() throws UnsupportedEncodingException {
final String charsetName = "UTF-8";
testNewString(charsetName);
final String expected = new String(BYTES_FIXTURE, charsetName);
final String actual = StringUtils.newStringUtf8(BYTES_FIXTURE);
Assert.assertEquals(expected, actual);
}
@Test
public void testEqualsString() {
Assert.assertTrue(StringUtils.equals(null, null));
Assert.assertFalse(StringUtils.equals("abc", null));
Assert.assertFalse(StringUtils.equals(null, "abc"));
Assert.assertTrue(StringUtils.equals("abc", "abc"));
Assert.assertFalse(StringUtils.equals("abc", "abcd"));
Assert.assertFalse(StringUtils.equals("abcd", "abc"));
Assert.assertFalse(StringUtils.equals("abc", "ABC"));
}
@Test
public void testEqualsCS1() {
Assert.assertFalse(StringUtils.equals(new StringBuilder("abc"), null));
Assert.assertFalse(StringUtils.equals(null, new StringBuilder("abc")));
Assert.assertTrue(StringUtils.equals(new StringBuilder("abc"), new StringBuilder("abc")));
Assert.assertFalse(StringUtils.equals(new StringBuilder("abc"), new StringBuilder("abcd")));
Assert.assertFalse(StringUtils.equals(new StringBuilder("abcd"), new StringBuilder("abc")));
Assert.assertFalse(StringUtils.equals(new StringBuilder("abc"), new StringBuilder("ABC")));
}
@Test
public void testEqualsCS2() {
Assert.assertTrue(StringUtils.equals("abc", new StringBuilder("abc")));
Assert.assertFalse(StringUtils.equals(new StringBuilder("abc"), "abcd"));
Assert.assertFalse(StringUtils.equals("abcd", new StringBuilder("abc")));
Assert.assertFalse(StringUtils.equals(new StringBuilder("abc"), "ABC"));
}
} | // You are a professional Java test case writer, please create a test case named `testEqualsCS1` for the issue `Codec-CODEC-231`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Codec-CODEC-231
//
// ## Issue-Title:
// StringUtils.equals(CharSequence cs1, CharSequence cs2) can fail with String Index OBE
//
// ## Issue-Description:
//
// StringUtils.equals(CharSequence cs1, CharSequence cs2) fails with String Index OBE if the two sequences are different lengths.
//
//
//
//
//
@Test
public void testEqualsCS1() {
| 230 | 18 | 222 | src/test/java/org/apache/commons/codec/binary/StringUtilsTest.java | src/test/java | ```markdown
## Issue-ID: Codec-CODEC-231
## Issue-Title:
StringUtils.equals(CharSequence cs1, CharSequence cs2) can fail with String Index OBE
## Issue-Description:
StringUtils.equals(CharSequence cs1, CharSequence cs2) fails with String Index OBE if the two sequences are different lengths.
```
You are a professional Java test case writer, please create a test case named `testEqualsCS1` for the issue `Codec-CODEC-231`, utilizing the provided issue report information and the following function signature.
```java
@Test
public void testEqualsCS1() {
```
| 222 | [
"org.apache.commons.codec.binary.StringUtils"
] | 9c7cf79b8f29e168d3699b330ceb3062fffd449b19b3c33f47d44e2c7307eb7e | @Test
public void testEqualsCS1() | // You are a professional Java test case writer, please create a test case named `testEqualsCS1` for the issue `Codec-CODEC-231`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Codec-CODEC-231
//
// ## Issue-Title:
// StringUtils.equals(CharSequence cs1, CharSequence cs2) can fail with String Index OBE
//
// ## Issue-Description:
//
// StringUtils.equals(CharSequence cs1, CharSequence cs2) fails with String Index OBE if the two sequences are different lengths.
//
//
//
//
//
| Codec | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.codec.binary;
import java.io.UnsupportedEncodingException;
import java.util.Arrays;
import org.junit.Assert;
import org.junit.Test;
/**
* Tests {@link StringUtils}
*
* @version $Id$
*/
public class StringUtilsTest {
private static final byte[] BYTES_FIXTURE = {'a','b','c'};
// This is valid input for UTF-16BE
private static final byte[] BYTES_FIXTURE_16BE = {0, 'a', 0, 'b', 0, 'c'};
// This is valid for UTF-16LE
private static final byte[] BYTES_FIXTURE_16LE = {'a', 0, 'b', 0, 'c', 0};
private static final String STRING_FIXTURE = "ABC";
/**
* We could make the constructor private but there does not seem to be a point to jumping through extra code hoops
* to restrict instantiation right now.
*/
@Test
public void testConstructor() {
new StringUtils();
}
@Test
public void testGetBytesIso8859_1() throws UnsupportedEncodingException {
final String charsetName = "ISO-8859-1";
testGetBytesUnchecked(charsetName);
final byte[] expected = STRING_FIXTURE.getBytes(charsetName);
final byte[] actual = StringUtils.getBytesIso8859_1(STRING_FIXTURE);
Assert.assertTrue(Arrays.equals(expected, actual));
}
private void testGetBytesUnchecked(final String charsetName) throws UnsupportedEncodingException {
final byte[] expected = STRING_FIXTURE.getBytes(charsetName);
final byte[] actual = StringUtils.getBytesUnchecked(STRING_FIXTURE, charsetName);
Assert.assertTrue(Arrays.equals(expected, actual));
}
@Test
public void testGetBytesUsAscii() throws UnsupportedEncodingException {
final String charsetName = "US-ASCII";
testGetBytesUnchecked(charsetName);
final byte[] expected = STRING_FIXTURE.getBytes(charsetName);
final byte[] actual = StringUtils.getBytesUsAscii(STRING_FIXTURE);
Assert.assertTrue(Arrays.equals(expected, actual));
}
@Test
public void testGetBytesUtf16() throws UnsupportedEncodingException {
final String charsetName = "UTF-16";
testGetBytesUnchecked(charsetName);
final byte[] expected = STRING_FIXTURE.getBytes(charsetName);
final byte[] actual = StringUtils.getBytesUtf16(STRING_FIXTURE);
Assert.assertTrue(Arrays.equals(expected, actual));
}
@Test
public void testGetBytesUtf16Be() throws UnsupportedEncodingException {
final String charsetName = "UTF-16BE";
testGetBytesUnchecked(charsetName);
final byte[] expected = STRING_FIXTURE.getBytes(charsetName);
final byte[] actual = StringUtils.getBytesUtf16Be(STRING_FIXTURE);
Assert.assertTrue(Arrays.equals(expected, actual));
}
@Test
public void testGetBytesUtf16Le() throws UnsupportedEncodingException {
final String charsetName = "UTF-16LE";
testGetBytesUnchecked(charsetName);
final byte[] expected = STRING_FIXTURE.getBytes(charsetName);
final byte[] actual = StringUtils.getBytesUtf16Le(STRING_FIXTURE);
Assert.assertTrue(Arrays.equals(expected, actual));
}
@Test
public void testGetBytesUtf8() throws UnsupportedEncodingException {
final String charsetName = "UTF-8";
testGetBytesUnchecked(charsetName);
final byte[] expected = STRING_FIXTURE.getBytes(charsetName);
final byte[] actual = StringUtils.getBytesUtf8(STRING_FIXTURE);
Assert.assertTrue(Arrays.equals(expected, actual));
}
@Test
public void testGetBytesUncheckedBadName() {
try {
StringUtils.getBytesUnchecked(STRING_FIXTURE, "UNKNOWN");
Assert.fail("Expected " + IllegalStateException.class.getName());
} catch (final IllegalStateException e) {
// Expected
}
}
@Test
public void testGetBytesUncheckedNullInput() {
Assert.assertNull(StringUtils.getBytesUnchecked(null, "UNKNOWN"));
}
private void testNewString(final String charsetName) throws UnsupportedEncodingException {
final String expected = new String(BYTES_FIXTURE, charsetName);
final String actual = StringUtils.newString(BYTES_FIXTURE, charsetName);
Assert.assertEquals(expected, actual);
}
@Test
public void testNewStringBadEnc() {
try {
StringUtils.newString(BYTES_FIXTURE, "UNKNOWN");
Assert.fail("Expected " + IllegalStateException.class.getName());
} catch (final IllegalStateException e) {
// Expected
}
}
@Test
public void testNewStringNullInput() {
Assert.assertNull(StringUtils.newString(null, "UNKNOWN"));
}
@Test
public void testNewStringNullInput_CODEC229() {
Assert.assertNull(StringUtils.newStringUtf8(null));
Assert.assertNull(StringUtils.newStringIso8859_1(null));
Assert.assertNull(StringUtils.newStringUsAscii(null));
Assert.assertNull(StringUtils.newStringUtf16(null));
Assert.assertNull(StringUtils.newStringUtf16Be(null));
Assert.assertNull(StringUtils.newStringUtf16Le(null));
}
@Test
public void testNewStringIso8859_1() throws UnsupportedEncodingException {
final String charsetName = "ISO-8859-1";
testNewString(charsetName);
final String expected = new String(BYTES_FIXTURE, charsetName);
final String actual = StringUtils.newStringIso8859_1(BYTES_FIXTURE);
Assert.assertEquals(expected, actual);
}
@Test
public void testNewStringUsAscii() throws UnsupportedEncodingException {
final String charsetName = "US-ASCII";
testNewString(charsetName);
final String expected = new String(BYTES_FIXTURE, charsetName);
final String actual = StringUtils.newStringUsAscii(BYTES_FIXTURE);
Assert.assertEquals(expected, actual);
}
@Test
public void testNewStringUtf16() throws UnsupportedEncodingException {
final String charsetName = "UTF-16";
testNewString(charsetName);
final String expected = new String(BYTES_FIXTURE, charsetName);
final String actual = StringUtils.newStringUtf16(BYTES_FIXTURE);
Assert.assertEquals(expected, actual);
}
@Test
public void testNewStringUtf16Be() throws UnsupportedEncodingException {
final String charsetName = "UTF-16BE";
testNewString(charsetName);
final String expected = new String(BYTES_FIXTURE_16BE, charsetName);
final String actual = StringUtils.newStringUtf16Be(BYTES_FIXTURE_16BE);
Assert.assertEquals(expected, actual);
}
@Test
public void testNewStringUtf16Le() throws UnsupportedEncodingException {
final String charsetName = "UTF-16LE";
testNewString(charsetName);
final String expected = new String(BYTES_FIXTURE_16LE, charsetName);
final String actual = StringUtils.newStringUtf16Le(BYTES_FIXTURE_16LE);
Assert.assertEquals(expected, actual);
}
@Test
public void testNewStringUtf8() throws UnsupportedEncodingException {
final String charsetName = "UTF-8";
testNewString(charsetName);
final String expected = new String(BYTES_FIXTURE, charsetName);
final String actual = StringUtils.newStringUtf8(BYTES_FIXTURE);
Assert.assertEquals(expected, actual);
}
@Test
public void testEqualsString() {
Assert.assertTrue(StringUtils.equals(null, null));
Assert.assertFalse(StringUtils.equals("abc", null));
Assert.assertFalse(StringUtils.equals(null, "abc"));
Assert.assertTrue(StringUtils.equals("abc", "abc"));
Assert.assertFalse(StringUtils.equals("abc", "abcd"));
Assert.assertFalse(StringUtils.equals("abcd", "abc"));
Assert.assertFalse(StringUtils.equals("abc", "ABC"));
}
@Test
public void testEqualsCS1() {
Assert.assertFalse(StringUtils.equals(new StringBuilder("abc"), null));
Assert.assertFalse(StringUtils.equals(null, new StringBuilder("abc")));
Assert.assertTrue(StringUtils.equals(new StringBuilder("abc"), new StringBuilder("abc")));
Assert.assertFalse(StringUtils.equals(new StringBuilder("abc"), new StringBuilder("abcd")));
Assert.assertFalse(StringUtils.equals(new StringBuilder("abcd"), new StringBuilder("abc")));
Assert.assertFalse(StringUtils.equals(new StringBuilder("abc"), new StringBuilder("ABC")));
}
@Test
public void testEqualsCS2() {
Assert.assertTrue(StringUtils.equals("abc", new StringBuilder("abc")));
Assert.assertFalse(StringUtils.equals(new StringBuilder("abc"), "abcd"));
Assert.assertFalse(StringUtils.equals("abcd", new StringBuilder("abc")));
Assert.assertFalse(StringUtils.equals(new StringBuilder("abc"), "ABC"));
}
} |
||
public void testGcd() {
int a = 30;
int b = 50;
int c = 77;
assertEquals(0, MathUtils.gcd(0, 0));
assertEquals(b, MathUtils.gcd(0, b));
assertEquals(a, MathUtils.gcd(a, 0));
assertEquals(b, MathUtils.gcd(0, -b));
assertEquals(a, MathUtils.gcd(-a, 0));
assertEquals(10, MathUtils.gcd(a, b));
assertEquals(10, MathUtils.gcd(-a, b));
assertEquals(10, MathUtils.gcd(a, -b));
assertEquals(10, MathUtils.gcd(-a, -b));
assertEquals(1, MathUtils.gcd(a, c));
assertEquals(1, MathUtils.gcd(-a, c));
assertEquals(1, MathUtils.gcd(a, -c));
assertEquals(1, MathUtils.gcd(-a, -c));
assertEquals(3 * (1<<15), MathUtils.gcd(3 * (1<<20), 9 * (1<<15)));
} | org.apache.commons.math.util.MathUtilsTest::testGcd | src/test/org/apache/commons/math/util/MathUtilsTest.java | 296 | src/test/org/apache/commons/math/util/MathUtilsTest.java | testGcd | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with this
* work for additional information regarding copyright ownership. The ASF
* licenses this file to You under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0 Unless required by applicable law
* or agreed to in writing, software distributed under the License is
* distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package org.apache.commons.math.util;
import java.math.BigDecimal;
import junit.framework.Test;
import junit.framework.TestCase;
import junit.framework.TestSuite;
import org.apache.commons.math.random.RandomDataImpl;
import org.apache.commons.math.TestUtils;
/**
* Test cases for the MathUtils class.
* @version $Revision$ $Date: 2007-08-16 15:36:33 -0500 (Thu, 16 Aug
* 2007) $
*/
public final class MathUtilsTest extends TestCase {
public MathUtilsTest(String name) {
super(name);
}
public static Test suite() {
TestSuite suite = new TestSuite(MathUtilsTest.class);
suite.setName("MathUtils Tests");
return suite;
}
/**
* Exact recursive implementation to test against
*/
private long binomialCoefficient(int n, int k) {
if ((n == k) || (k == 0)) {
return 1;
}
if ((k == 1) || (k == n - 1)) {
return n;
}
return binomialCoefficient(n - 1, k - 1) + binomialCoefficient(n - 1, k);
}
/**
* Exact direct multiplication implementation to test against
*/
private long factorial(int n) {
long result = 1;
for (int i = 2; i <= n; i++) {
result *= i;
}
return result;
}
/** Verify that b(0,0) = 1 */
public void test0Choose0() {
assertEquals(MathUtils.binomialCoefficientDouble(0, 0), 1d, 0);
assertEquals(MathUtils.binomialCoefficientLog(0, 0), 0d, 0);
assertEquals(MathUtils.binomialCoefficient(0, 0), 1);
}
public void testAddAndCheck() {
int big = Integer.MAX_VALUE;
int bigNeg = Integer.MIN_VALUE;
assertEquals(big, MathUtils.addAndCheck(big, 0));
try {
MathUtils.addAndCheck(big, 1);
fail("Expecting ArithmeticException");
} catch (ArithmeticException ex) {
}
try {
MathUtils.addAndCheck(bigNeg, -1);
fail("Expecting ArithmeticException");
} catch (ArithmeticException ex) {
}
}
public void testAddAndCheckLong() {
long max = Long.MAX_VALUE;
long min = Long.MIN_VALUE;
assertEquals(max, MathUtils.addAndCheck(max, 0L));
assertEquals(min, MathUtils.addAndCheck(min, 0L));
assertEquals(max, MathUtils.addAndCheck(0L, max));
assertEquals(min, MathUtils.addAndCheck(0L, min));
assertEquals(1, MathUtils.addAndCheck(-1L, 2L));
assertEquals(1, MathUtils.addAndCheck(2L, -1L));
testAddAndCheckLongFailure(max, 1L);
testAddAndCheckLongFailure(min, -1L);
testAddAndCheckLongFailure(1L, max);
testAddAndCheckLongFailure(-1L, min);
}
private void testAddAndCheckLongFailure(long a, long b) {
try {
MathUtils.addAndCheck(a, b);
fail("Expecting ArithmeticException");
} catch (ArithmeticException ex) {
// success
}
}
public void testBinomialCoefficient() {
long[] bcoef5 = {
1,
5,
10,
10,
5,
1 };
long[] bcoef6 = {
1,
6,
15,
20,
15,
6,
1 };
for (int i = 0; i < 6; i++) {
assertEquals("5 choose " + i, bcoef5[i], MathUtils.binomialCoefficient(5, i));
}
for (int i = 0; i < 7; i++) {
assertEquals("6 choose " + i, bcoef6[i], MathUtils.binomialCoefficient(6, i));
}
for (int n = 1; n < 10; n++) {
for (int k = 0; k <= n; k++) {
assertEquals(n + " choose " + k, binomialCoefficient(n, k), MathUtils.binomialCoefficient(n, k));
assertEquals(n + " choose " + k, (double)binomialCoefficient(n, k), MathUtils.binomialCoefficientDouble(n, k), Double.MIN_VALUE);
assertEquals(n + " choose " + k, Math.log((double)binomialCoefficient(n, k)), MathUtils.binomialCoefficientLog(n, k), 10E-12);
}
}
/*
* Takes a long time for recursion to unwind, but succeeds and yields
* exact value = 2,333,606,220
* assertEquals(MathUtils.binomialCoefficient(34,17),
* binomialCoefficient(34,17));
*/
}
public void testBinomialCoefficientFail() {
try {
MathUtils.binomialCoefficient(4, 5);
fail("expecting IllegalArgumentException");
} catch (IllegalArgumentException ex) {
;
}
try {
MathUtils.binomialCoefficientDouble(4, 5);
fail("expecting IllegalArgumentException");
} catch (IllegalArgumentException ex) {
;
}
try {
MathUtils.binomialCoefficientLog(4, 5);
fail("expecting IllegalArgumentException");
} catch (IllegalArgumentException ex) {
;
}
try {
MathUtils.binomialCoefficient(67, 34);
fail("expecting ArithmeticException");
} catch (ArithmeticException ex) {
;
}
double x = MathUtils.binomialCoefficientDouble(1030, 515);
assertTrue("expecting infinite binomial coefficient", Double.isInfinite(x));
}
public void testCosh() {
double x = 3.0;
double expected = 10.06766;
assertEquals(expected, MathUtils.cosh(x), 1.0e-5);
}
public void testCoshNaN() {
assertTrue(Double.isNaN(MathUtils.cosh(Double.NaN)));
}
public void testEquals() {
double[] testArray = {
Double.NaN,
Double.POSITIVE_INFINITY,
Double.NEGATIVE_INFINITY,
1d,
0d };
for (int i = 0; i < testArray.length; i++) {
for (int j = 0; j < testArray.length; j++) {
if (i == j) {
assertTrue(MathUtils.equals(testArray[i], testArray[j]));
assertTrue(MathUtils.equals(testArray[j], testArray[i]));
} else {
assertTrue(!MathUtils.equals(testArray[i], testArray[j]));
assertTrue(!MathUtils.equals(testArray[j], testArray[i]));
}
}
}
}
public void testArrayEquals() {
assertFalse(MathUtils.equals(new double[] { 1d }, null));
assertFalse(MathUtils.equals(null, new double[] { 1d }));
assertTrue(MathUtils.equals((double[]) null, (double[]) null));
assertFalse(MathUtils.equals(new double[] { 1d }, new double[0]));
assertTrue(MathUtils.equals(new double[] { 1d }, new double[] { 1d }));
assertTrue(MathUtils.equals(new double[] {
Double.NaN, Double.POSITIVE_INFINITY,
Double.NEGATIVE_INFINITY, 1d, 0d
}, new double[] {
Double.NaN, Double.POSITIVE_INFINITY,
Double.NEGATIVE_INFINITY, 1d, 0d
}));
assertFalse(MathUtils.equals(new double[] { Double.POSITIVE_INFINITY },
new double[] { Double.NEGATIVE_INFINITY }));
assertFalse(MathUtils.equals(new double[] { 1d },
new double[] { MathUtils.nextAfter(1d, 2d) }));
}
public void testFactorial() {
for (int i = 1; i < 10; i++) {
assertEquals(i + "! ", factorial(i), MathUtils.factorial(i));
assertEquals(i + "! ", (double)factorial(i), MathUtils.factorialDouble(i), Double.MIN_VALUE);
assertEquals(i + "! ", Math.log((double)factorial(i)), MathUtils.factorialLog(i), 10E-12);
}
assertEquals("0", 1, MathUtils.factorial(0));
assertEquals("0", 1.0d, MathUtils.factorialDouble(0), 1E-14);
assertEquals("0", 0.0d, MathUtils.factorialLog(0), 1E-14);
}
public void testFactorialFail() {
try {
MathUtils.factorial(-1);
fail("expecting IllegalArgumentException");
} catch (IllegalArgumentException ex) {
;
}
try {
MathUtils.factorialDouble(-1);
fail("expecting IllegalArgumentException");
} catch (IllegalArgumentException ex) {
;
}
try {
MathUtils.factorialLog(-1);
fail("expecting IllegalArgumentException");
} catch (IllegalArgumentException ex) {
;
}
try {
MathUtils.factorial(21);
fail("expecting ArithmeticException");
} catch (ArithmeticException ex) {
;
}
assertTrue("expecting infinite factorial value", Double.isInfinite(MathUtils.factorialDouble(171)));
}
public void testGcd() {
int a = 30;
int b = 50;
int c = 77;
assertEquals(0, MathUtils.gcd(0, 0));
assertEquals(b, MathUtils.gcd(0, b));
assertEquals(a, MathUtils.gcd(a, 0));
assertEquals(b, MathUtils.gcd(0, -b));
assertEquals(a, MathUtils.gcd(-a, 0));
assertEquals(10, MathUtils.gcd(a, b));
assertEquals(10, MathUtils.gcd(-a, b));
assertEquals(10, MathUtils.gcd(a, -b));
assertEquals(10, MathUtils.gcd(-a, -b));
assertEquals(1, MathUtils.gcd(a, c));
assertEquals(1, MathUtils.gcd(-a, c));
assertEquals(1, MathUtils.gcd(a, -c));
assertEquals(1, MathUtils.gcd(-a, -c));
assertEquals(3 * (1<<15), MathUtils.gcd(3 * (1<<20), 9 * (1<<15)));
}
public void testHash() {
double[] testArray = {
Double.NaN,
Double.POSITIVE_INFINITY,
Double.NEGATIVE_INFINITY,
1d,
0d,
1E-14,
(1 + 1E-14),
Double.MIN_VALUE,
Double.MAX_VALUE };
for (int i = 0; i < testArray.length; i++) {
for (int j = 0; j < testArray.length; j++) {
if (i == j) {
assertEquals(MathUtils.hash(testArray[i]), MathUtils.hash(testArray[j]));
assertEquals(MathUtils.hash(testArray[j]), MathUtils.hash(testArray[i]));
} else {
assertTrue(MathUtils.hash(testArray[i]) != MathUtils.hash(testArray[j]));
assertTrue(MathUtils.hash(testArray[j]) != MathUtils.hash(testArray[i]));
}
}
}
}
public void testArrayHash() {
assertEquals(0, MathUtils.hash((double[]) null));
assertEquals(MathUtils.hash(new double[] {
Double.NaN, Double.POSITIVE_INFINITY,
Double.NEGATIVE_INFINITY, 1d, 0d
}),
MathUtils.hash(new double[] {
Double.NaN, Double.POSITIVE_INFINITY,
Double.NEGATIVE_INFINITY, 1d, 0d
}));
assertFalse(MathUtils.hash(new double[] { 1d }) ==
MathUtils.hash(new double[] { MathUtils.nextAfter(1d, 2d) }));
assertFalse(MathUtils.hash(new double[] { 1d }) ==
MathUtils.hash(new double[] { 1d, 1d }));
}
/**
* Make sure that permuted arrays do not hash to the same value.
*/
public void testPermutedArrayHash() {
double[] original = new double[10];
double[] permuted = new double[10];
RandomDataImpl random = new RandomDataImpl();
// Generate 10 distinct random values
for (int i = 0; i < 10; i++) {
original[i] = random.nextUniform((double)i + 0.5, (double)i + 0.75);
}
// Generate a random permutation, making sure it is not the identity
boolean isIdentity = true;
do {
int[] permutation = random.nextPermutation(10, 10);
for (int i = 0; i < 10; i++) {
if (i != permutation[i]) {
isIdentity = false;
}
permuted[i] = original[permutation[i]];
}
} while (isIdentity);
// Verify that permuted array has different hash
assertFalse(MathUtils.hash(original) == MathUtils.hash(permuted));
}
public void testIndicatorByte() {
assertEquals((byte)1, MathUtils.indicator((byte)2));
assertEquals((byte)1, MathUtils.indicator((byte)0));
assertEquals((byte)(-1), MathUtils.indicator((byte)(-2)));
}
public void testIndicatorDouble() {
double delta = 0.0;
assertEquals(1.0, MathUtils.indicator(2.0), delta);
assertEquals(1.0, MathUtils.indicator(0.0), delta);
assertEquals(-1.0, MathUtils.indicator(-2.0), delta);
}
public void testIndicatorFloat() {
float delta = 0.0F;
assertEquals(1.0F, MathUtils.indicator(2.0F), delta);
assertEquals(1.0F, MathUtils.indicator(0.0F), delta);
assertEquals(-1.0F, MathUtils.indicator(-2.0F), delta);
}
public void testIndicatorInt() {
assertEquals((int)1, MathUtils.indicator((int)(2)));
assertEquals((int)1, MathUtils.indicator((int)(0)));
assertEquals((int)(-1), MathUtils.indicator((int)(-2)));
}
public void testIndicatorLong() {
assertEquals(1L, MathUtils.indicator(2L));
assertEquals(1L, MathUtils.indicator(0L));
assertEquals(-1L, MathUtils.indicator(-2L));
}
public void testIndicatorShort() {
assertEquals((short)1, MathUtils.indicator((short)2));
assertEquals((short)1, MathUtils.indicator((short)0));
assertEquals((short)(-1), MathUtils.indicator((short)(-2)));
}
public void testLcm() {
int a = 30;
int b = 50;
int c = 77;
assertEquals(0, MathUtils.lcm(0, b));
assertEquals(0, MathUtils.lcm(a, 0));
assertEquals(b, MathUtils.lcm(1, b));
assertEquals(a, MathUtils.lcm(a, 1));
assertEquals(150, MathUtils.lcm(a, b));
assertEquals(150, MathUtils.lcm(-a, b));
assertEquals(150, MathUtils.lcm(a, -b));
assertEquals(2310, MathUtils.lcm(a, c));
try {
MathUtils.lcm(Integer.MAX_VALUE, Integer.MAX_VALUE - 1);
fail("Expecting ArithmeticException");
} catch (ArithmeticException ex) {
// expected
}
}
public void testLog() {
assertEquals(2.0, MathUtils.log(2, 4), 0);
assertEquals(3.0, MathUtils.log(2, 8), 0);
assertTrue(Double.isNaN(MathUtils.log(-1, 1)));
assertTrue(Double.isNaN(MathUtils.log(1, -1)));
assertTrue(Double.isNaN(MathUtils.log(0, 0)));
assertEquals(0, MathUtils.log(0, 10), 0);
assertEquals(Double.NEGATIVE_INFINITY, MathUtils.log(10, 0), 0);
}
public void testMulAndCheck() {
int big = Integer.MAX_VALUE;
int bigNeg = Integer.MIN_VALUE;
assertEquals(big, MathUtils.mulAndCheck(big, 1));
try {
MathUtils.mulAndCheck(big, 2);
fail("Expecting ArithmeticException");
} catch (ArithmeticException ex) {
}
try {
MathUtils.mulAndCheck(bigNeg, 2);
fail("Expecting ArithmeticException");
} catch (ArithmeticException ex) {
}
}
public void testMulAndCheckLong() {
long max = Long.MAX_VALUE;
long min = Long.MIN_VALUE;
assertEquals(max, MathUtils.mulAndCheck(max, 1L));
assertEquals(min, MathUtils.mulAndCheck(min, 1L));
assertEquals(0L, MathUtils.mulAndCheck(max, 0L));
assertEquals(0L, MathUtils.mulAndCheck(min, 0L));
assertEquals(max, MathUtils.mulAndCheck(1L, max));
assertEquals(min, MathUtils.mulAndCheck(1L, min));
assertEquals(0L, MathUtils.mulAndCheck(0L, max));
assertEquals(0L, MathUtils.mulAndCheck(0L, min));
testMulAndCheckLongFailure(max, 2L);
testMulAndCheckLongFailure(2L, max);
testMulAndCheckLongFailure(min, 2L);
testMulAndCheckLongFailure(2L, min);
testMulAndCheckLongFailure(min, -1L);
testMulAndCheckLongFailure(-1L, min);
}
private void testMulAndCheckLongFailure(long a, long b) {
try {
MathUtils.mulAndCheck(a, b);
fail("Expecting ArithmeticException");
} catch (ArithmeticException ex) {
// success
}
}
public void testNextAfter() {
// 0x402fffffffffffff 0x404123456789abcd -> 4030000000000000
assertEquals(16.0, MathUtils.nextAfter(15.999999999999998, 34.27555555555555), 0.0);
// 0xc02fffffffffffff 0x404123456789abcd -> c02ffffffffffffe
assertEquals(-15.999999999999996, MathUtils.nextAfter(-15.999999999999998, 34.27555555555555), 0.0);
// 0x402fffffffffffff 0x400123456789abcd -> 402ffffffffffffe
assertEquals(15.999999999999996, MathUtils.nextAfter(15.999999999999998, 2.142222222222222), 0.0);
// 0xc02fffffffffffff 0x400123456789abcd -> c02ffffffffffffe
assertEquals(-15.999999999999996, MathUtils.nextAfter(-15.999999999999998, 2.142222222222222), 0.0);
// 0x4020000000000000 0x404123456789abcd -> 4020000000000001
assertEquals(8.000000000000002, MathUtils.nextAfter(8.0, 34.27555555555555), 0.0);
// 0xc020000000000000 0x404123456789abcd -> c01fffffffffffff
assertEquals(-7.999999999999999, MathUtils.nextAfter(-8.0, 34.27555555555555), 0.0);
// 0x4020000000000000 0x400123456789abcd -> 401fffffffffffff
assertEquals(7.999999999999999, MathUtils.nextAfter(8.0, 2.142222222222222), 0.0);
// 0xc020000000000000 0x400123456789abcd -> c01fffffffffffff
assertEquals(-7.999999999999999, MathUtils.nextAfter(-8.0, 2.142222222222222), 0.0);
// 0x3f2e43753d36a223 0x3f2e43753d36a224 -> 3f2e43753d36a224
assertEquals(2.308922399667661E-4, MathUtils.nextAfter(2.3089223996676606E-4, 2.308922399667661E-4), 0.0);
// 0x3f2e43753d36a223 0x3f2e43753d36a223 -> 3f2e43753d36a224
assertEquals(2.308922399667661E-4, MathUtils.nextAfter(2.3089223996676606E-4, 2.3089223996676606E-4), 0.0);
// 0x3f2e43753d36a223 0x3f2e43753d36a222 -> 3f2e43753d36a222
assertEquals(2.3089223996676603E-4, MathUtils.nextAfter(2.3089223996676606E-4, 2.3089223996676603E-4), 0.0);
// 0x3f2e43753d36a223 0xbf2e43753d36a224 -> 3f2e43753d36a222
assertEquals(2.3089223996676603E-4, MathUtils.nextAfter(2.3089223996676606E-4, -2.308922399667661E-4), 0.0);
// 0x3f2e43753d36a223 0xbf2e43753d36a223 -> 3f2e43753d36a222
assertEquals(2.3089223996676603E-4, MathUtils.nextAfter(2.3089223996676606E-4, -2.3089223996676606E-4), 0.0);
// 0x3f2e43753d36a223 0xbf2e43753d36a222 -> 3f2e43753d36a222
assertEquals(2.3089223996676603E-4, MathUtils.nextAfter(2.3089223996676606E-4, -2.3089223996676603E-4), 0.0);
// 0xbf2e43753d36a223 0x3f2e43753d36a224 -> bf2e43753d36a222
assertEquals(-2.3089223996676603E-4, MathUtils.nextAfter(-2.3089223996676606E-4, 2.308922399667661E-4), 0.0);
// 0xbf2e43753d36a223 0x3f2e43753d36a223 -> bf2e43753d36a222
assertEquals(-2.3089223996676603E-4, MathUtils.nextAfter(-2.3089223996676606E-4, 2.3089223996676606E-4), 0.0);
// 0xbf2e43753d36a223 0x3f2e43753d36a222 -> bf2e43753d36a222
assertEquals(-2.3089223996676603E-4, MathUtils.nextAfter(-2.3089223996676606E-4, 2.3089223996676603E-4), 0.0);
// 0xbf2e43753d36a223 0xbf2e43753d36a224 -> bf2e43753d36a224
assertEquals(-2.308922399667661E-4, MathUtils.nextAfter(-2.3089223996676606E-4, -2.308922399667661E-4), 0.0);
// 0xbf2e43753d36a223 0xbf2e43753d36a223 -> bf2e43753d36a224
assertEquals(-2.308922399667661E-4, MathUtils.nextAfter(-2.3089223996676606E-4, -2.3089223996676606E-4), 0.0);
// 0xbf2e43753d36a223 0xbf2e43753d36a222 -> bf2e43753d36a222
assertEquals(-2.3089223996676603E-4, MathUtils.nextAfter(-2.3089223996676606E-4, -2.3089223996676603E-4), 0.0);
}
public void testNextAfterSpecialCases() {
assertTrue(Double.isInfinite(MathUtils.nextAfter(Double.NEGATIVE_INFINITY, 0)));
assertTrue(Double.isInfinite(MathUtils.nextAfter(Double.POSITIVE_INFINITY, 0)));
assertTrue(Double.isNaN(MathUtils.nextAfter(Double.NaN, 0)));
assertTrue(Double.isInfinite(MathUtils.nextAfter(Double.MAX_VALUE, Double.POSITIVE_INFINITY)));
assertTrue(Double.isInfinite(MathUtils.nextAfter(-Double.MAX_VALUE, Double.NEGATIVE_INFINITY)));
assertEquals(Double.MIN_VALUE, MathUtils.nextAfter(0, 1), 0);
assertEquals(-Double.MIN_VALUE, MathUtils.nextAfter(0, -1), 0);
assertEquals(0, MathUtils.nextAfter(Double.MIN_VALUE, -1), 0);
assertEquals(0, MathUtils.nextAfter(-Double.MIN_VALUE, 1), 0);
}
public void testScalb() {
assertEquals( 0.0, MathUtils.scalb(0.0, 5), 1.0e-15);
assertEquals(32.0, MathUtils.scalb(1.0, 5), 1.0e-15);
assertEquals(1.0 / 32.0, MathUtils.scalb(1.0, -5), 1.0e-15);
assertEquals(Math.PI, MathUtils.scalb(Math.PI, 0), 1.0e-15);
assertTrue(Double.isInfinite(MathUtils.scalb(Double.POSITIVE_INFINITY, 1)));
assertTrue(Double.isInfinite(MathUtils.scalb(Double.NEGATIVE_INFINITY, 1)));
assertTrue(Double.isNaN(MathUtils.scalb(Double.NaN, 1)));
}
public void testNormalizeAngle() {
for (double a = -15.0; a <= 15.0; a += 0.1) {
for (double b = -15.0; b <= 15.0; b += 0.2) {
double c = MathUtils.normalizeAngle(a, b);
assertTrue((b - Math.PI) <= c);
assertTrue(c <= (b + Math.PI));
double twoK = Math.rint((a - c) / Math.PI);
assertEquals(c, a - twoK * Math.PI, 1.0e-14);
}
}
}
public void testRoundDouble() {
double x = 1.234567890;
assertEquals(1.23, MathUtils.round(x, 2), 0.0);
assertEquals(1.235, MathUtils.round(x, 3), 0.0);
assertEquals(1.2346, MathUtils.round(x, 4), 0.0);
// JIRA MATH-151
assertEquals(39.25, MathUtils.round(39.245, 2), 0.0);
assertEquals(39.24, MathUtils.round(39.245, 2, BigDecimal.ROUND_DOWN), 0.0);
double xx = 39.0;
xx = xx + 245d / 1000d;
assertEquals(39.25, MathUtils.round(xx, 2), 0.0);
// BZ 35904
assertEquals(30.1d, MathUtils.round(30.095d, 2), 0.0d);
assertEquals(30.1d, MathUtils.round(30.095d, 1), 0.0d);
assertEquals(33.1d, MathUtils.round(33.095d, 1), 0.0d);
assertEquals(33.1d, MathUtils.round(33.095d, 2), 0.0d);
assertEquals(50.09d, MathUtils.round(50.085d, 2), 0.0d);
assertEquals(50.19d, MathUtils.round(50.185d, 2), 0.0d);
assertEquals(50.01d, MathUtils.round(50.005d, 2), 0.0d);
assertEquals(30.01d, MathUtils.round(30.005d, 2), 0.0d);
assertEquals(30.65d, MathUtils.round(30.645d, 2), 0.0d);
assertEquals(1.24, MathUtils.round(x, 2, BigDecimal.ROUND_CEILING), 0.0);
assertEquals(1.235, MathUtils.round(x, 3, BigDecimal.ROUND_CEILING), 0.0);
assertEquals(1.2346, MathUtils.round(x, 4, BigDecimal.ROUND_CEILING), 0.0);
assertEquals(-1.23, MathUtils.round(-x, 2, BigDecimal.ROUND_CEILING), 0.0);
assertEquals(-1.234, MathUtils.round(-x, 3, BigDecimal.ROUND_CEILING), 0.0);
assertEquals(-1.2345, MathUtils.round(-x, 4, BigDecimal.ROUND_CEILING), 0.0);
assertEquals(1.23, MathUtils.round(x, 2, BigDecimal.ROUND_DOWN), 0.0);
assertEquals(1.234, MathUtils.round(x, 3, BigDecimal.ROUND_DOWN), 0.0);
assertEquals(1.2345, MathUtils.round(x, 4, BigDecimal.ROUND_DOWN), 0.0);
assertEquals(-1.23, MathUtils.round(-x, 2, BigDecimal.ROUND_DOWN), 0.0);
assertEquals(-1.234, MathUtils.round(-x, 3, BigDecimal.ROUND_DOWN), 0.0);
assertEquals(-1.2345, MathUtils.round(-x, 4, BigDecimal.ROUND_DOWN), 0.0);
assertEquals(1.23, MathUtils.round(x, 2, BigDecimal.ROUND_FLOOR), 0.0);
assertEquals(1.234, MathUtils.round(x, 3, BigDecimal.ROUND_FLOOR), 0.0);
assertEquals(1.2345, MathUtils.round(x, 4, BigDecimal.ROUND_FLOOR), 0.0);
assertEquals(-1.24, MathUtils.round(-x, 2, BigDecimal.ROUND_FLOOR), 0.0);
assertEquals(-1.235, MathUtils.round(-x, 3, BigDecimal.ROUND_FLOOR), 0.0);
assertEquals(-1.2346, MathUtils.round(-x, 4, BigDecimal.ROUND_FLOOR), 0.0);
assertEquals(1.23, MathUtils.round(x, 2, BigDecimal.ROUND_HALF_DOWN), 0.0);
assertEquals(1.235, MathUtils.round(x, 3, BigDecimal.ROUND_HALF_DOWN), 0.0);
assertEquals(1.2346, MathUtils.round(x, 4, BigDecimal.ROUND_HALF_DOWN), 0.0);
assertEquals(-1.23, MathUtils.round(-x, 2, BigDecimal.ROUND_HALF_DOWN), 0.0);
assertEquals(-1.235, MathUtils.round(-x, 3, BigDecimal.ROUND_HALF_DOWN), 0.0);
assertEquals(-1.2346, MathUtils.round(-x, 4, BigDecimal.ROUND_HALF_DOWN), 0.0);
assertEquals(1.234, MathUtils.round(1.2345, 3, BigDecimal.ROUND_HALF_DOWN), 0.0);
assertEquals(-1.234, MathUtils.round(-1.2345, 3, BigDecimal.ROUND_HALF_DOWN), 0.0);
assertEquals(1.23, MathUtils.round(x, 2, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(1.235, MathUtils.round(x, 3, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(1.2346, MathUtils.round(x, 4, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(-1.23, MathUtils.round(-x, 2, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(-1.235, MathUtils.round(-x, 3, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(-1.2346, MathUtils.round(-x, 4, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(1.234, MathUtils.round(1.2345, 3, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(-1.234, MathUtils.round(-1.2345, 3, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(1.236, MathUtils.round(1.2355, 3, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(-1.236, MathUtils.round(-1.2355, 3, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(1.23, MathUtils.round(x, 2, BigDecimal.ROUND_HALF_UP), 0.0);
assertEquals(1.235, MathUtils.round(x, 3, BigDecimal.ROUND_HALF_UP), 0.0);
assertEquals(1.2346, MathUtils.round(x, 4, BigDecimal.ROUND_HALF_UP), 0.0);
assertEquals(-1.23, MathUtils.round(-x, 2, BigDecimal.ROUND_HALF_UP), 0.0);
assertEquals(-1.235, MathUtils.round(-x, 3, BigDecimal.ROUND_HALF_UP), 0.0);
assertEquals(-1.2346, MathUtils.round(-x, 4, BigDecimal.ROUND_HALF_UP), 0.0);
assertEquals(1.235, MathUtils.round(1.2345, 3, BigDecimal.ROUND_HALF_UP), 0.0);
assertEquals(-1.235, MathUtils.round(-1.2345, 3, BigDecimal.ROUND_HALF_UP), 0.0);
assertEquals(-1.23, MathUtils.round(-1.23, 2, BigDecimal.ROUND_UNNECESSARY), 0.0);
assertEquals(1.23, MathUtils.round(1.23, 2, BigDecimal.ROUND_UNNECESSARY), 0.0);
try {
MathUtils.round(1.234, 2, BigDecimal.ROUND_UNNECESSARY);
fail();
} catch (ArithmeticException ex) {
// success
}
assertEquals(1.24, MathUtils.round(x, 2, BigDecimal.ROUND_UP), 0.0);
assertEquals(1.235, MathUtils.round(x, 3, BigDecimal.ROUND_UP), 0.0);
assertEquals(1.2346, MathUtils.round(x, 4, BigDecimal.ROUND_UP), 0.0);
assertEquals(-1.24, MathUtils.round(-x, 2, BigDecimal.ROUND_UP), 0.0);
assertEquals(-1.235, MathUtils.round(-x, 3, BigDecimal.ROUND_UP), 0.0);
assertEquals(-1.2346, MathUtils.round(-x, 4, BigDecimal.ROUND_UP), 0.0);
try {
MathUtils.round(1.234, 2, 1923);
fail();
} catch (IllegalArgumentException ex) {
// success
}
// MATH-151
assertEquals(39.25, MathUtils.round(39.245, 2, BigDecimal.ROUND_HALF_UP), 0.0);
// special values
TestUtils.assertEquals(Double.NaN, MathUtils.round(Double.NaN, 2), 0.0);
assertEquals(0.0, MathUtils.round(0.0, 2), 0.0);
assertEquals(Double.POSITIVE_INFINITY, MathUtils.round(Double.POSITIVE_INFINITY, 2), 0.0);
assertEquals(Double.NEGATIVE_INFINITY, MathUtils.round(Double.NEGATIVE_INFINITY, 2), 0.0);
}
public void testRoundFloat() {
float x = 1.234567890f;
assertEquals(1.23f, MathUtils.round(x, 2), 0.0);
assertEquals(1.235f, MathUtils.round(x, 3), 0.0);
assertEquals(1.2346f, MathUtils.round(x, 4), 0.0);
// BZ 35904
assertEquals(30.1f, MathUtils.round(30.095f, 2), 0.0f);
assertEquals(30.1f, MathUtils.round(30.095f, 1), 0.0f);
assertEquals(50.09f, MathUtils.round(50.085f, 2), 0.0f);
assertEquals(50.19f, MathUtils.round(50.185f, 2), 0.0f);
assertEquals(50.01f, MathUtils.round(50.005f, 2), 0.0f);
assertEquals(30.01f, MathUtils.round(30.005f, 2), 0.0f);
assertEquals(30.65f, MathUtils.round(30.645f, 2), 0.0f);
assertEquals(1.24f, MathUtils.round(x, 2, BigDecimal.ROUND_CEILING), 0.0);
assertEquals(1.235f, MathUtils.round(x, 3, BigDecimal.ROUND_CEILING), 0.0);
assertEquals(1.2346f, MathUtils.round(x, 4, BigDecimal.ROUND_CEILING), 0.0);
assertEquals(-1.23f, MathUtils.round(-x, 2, BigDecimal.ROUND_CEILING), 0.0);
assertEquals(-1.234f, MathUtils.round(-x, 3, BigDecimal.ROUND_CEILING), 0.0);
assertEquals(-1.2345f, MathUtils.round(-x, 4, BigDecimal.ROUND_CEILING), 0.0);
assertEquals(1.23f, MathUtils.round(x, 2, BigDecimal.ROUND_DOWN), 0.0);
assertEquals(1.234f, MathUtils.round(x, 3, BigDecimal.ROUND_DOWN), 0.0);
assertEquals(1.2345f, MathUtils.round(x, 4, BigDecimal.ROUND_DOWN), 0.0);
assertEquals(-1.23f, MathUtils.round(-x, 2, BigDecimal.ROUND_DOWN), 0.0);
assertEquals(-1.234f, MathUtils.round(-x, 3, BigDecimal.ROUND_DOWN), 0.0);
assertEquals(-1.2345f, MathUtils.round(-x, 4, BigDecimal.ROUND_DOWN), 0.0);
assertEquals(1.23f, MathUtils.round(x, 2, BigDecimal.ROUND_FLOOR), 0.0);
assertEquals(1.234f, MathUtils.round(x, 3, BigDecimal.ROUND_FLOOR), 0.0);
assertEquals(1.2345f, MathUtils.round(x, 4, BigDecimal.ROUND_FLOOR), 0.0);
assertEquals(-1.24f, MathUtils.round(-x, 2, BigDecimal.ROUND_FLOOR), 0.0);
assertEquals(-1.235f, MathUtils.round(-x, 3, BigDecimal.ROUND_FLOOR), 0.0);
assertEquals(-1.2346f, MathUtils.round(-x, 4, BigDecimal.ROUND_FLOOR), 0.0);
assertEquals(1.23f, MathUtils.round(x, 2, BigDecimal.ROUND_HALF_DOWN), 0.0);
assertEquals(1.235f, MathUtils.round(x, 3, BigDecimal.ROUND_HALF_DOWN), 0.0);
assertEquals(1.2346f, MathUtils.round(x, 4, BigDecimal.ROUND_HALF_DOWN), 0.0);
assertEquals(-1.23f, MathUtils.round(-x, 2, BigDecimal.ROUND_HALF_DOWN), 0.0);
assertEquals(-1.235f, MathUtils.round(-x, 3, BigDecimal.ROUND_HALF_DOWN), 0.0);
assertEquals(-1.2346f, MathUtils.round(-x, 4, BigDecimal.ROUND_HALF_DOWN), 0.0);
assertEquals(1.234f, MathUtils.round(1.2345f, 3, BigDecimal.ROUND_HALF_DOWN), 0.0);
assertEquals(-1.234f, MathUtils.round(-1.2345f, 3, BigDecimal.ROUND_HALF_DOWN), 0.0);
assertEquals(1.23f, MathUtils.round(x, 2, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(1.235f, MathUtils.round(x, 3, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(1.2346f, MathUtils.round(x, 4, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(-1.23f, MathUtils.round(-x, 2, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(-1.235f, MathUtils.round(-x, 3, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(-1.2346f, MathUtils.round(-x, 4, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(1.234f, MathUtils.round(1.2345f, 3, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(-1.234f, MathUtils.round(-1.2345f, 3, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(1.236f, MathUtils.round(1.2355f, 3, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(-1.236f, MathUtils.round(-1.2355f, 3, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(1.23f, MathUtils.round(x, 2, BigDecimal.ROUND_HALF_UP), 0.0);
assertEquals(1.235f, MathUtils.round(x, 3, BigDecimal.ROUND_HALF_UP), 0.0);
assertEquals(1.2346f, MathUtils.round(x, 4, BigDecimal.ROUND_HALF_UP), 0.0);
assertEquals(-1.23f, MathUtils.round(-x, 2, BigDecimal.ROUND_HALF_UP), 0.0);
assertEquals(-1.235f, MathUtils.round(-x, 3, BigDecimal.ROUND_HALF_UP), 0.0);
assertEquals(-1.2346f, MathUtils.round(-x, 4, BigDecimal.ROUND_HALF_UP), 0.0);
assertEquals(1.235f, MathUtils.round(1.2345f, 3, BigDecimal.ROUND_HALF_UP), 0.0);
assertEquals(-1.235f, MathUtils.round(-1.2345f, 3, BigDecimal.ROUND_HALF_UP), 0.0);
assertEquals(-1.23f, MathUtils.round(-1.23f, 2, BigDecimal.ROUND_UNNECESSARY), 0.0);
assertEquals(1.23f, MathUtils.round(1.23f, 2, BigDecimal.ROUND_UNNECESSARY), 0.0);
try {
MathUtils.round(1.234f, 2, BigDecimal.ROUND_UNNECESSARY);
fail();
} catch (ArithmeticException ex) {
// success
}
assertEquals(1.24f, MathUtils.round(x, 2, BigDecimal.ROUND_UP), 0.0);
assertEquals(1.235f, MathUtils.round(x, 3, BigDecimal.ROUND_UP), 0.0);
assertEquals(1.2346f, MathUtils.round(x, 4, BigDecimal.ROUND_UP), 0.0);
assertEquals(-1.24f, MathUtils.round(-x, 2, BigDecimal.ROUND_UP), 0.0);
assertEquals(-1.235f, MathUtils.round(-x, 3, BigDecimal.ROUND_UP), 0.0);
assertEquals(-1.2346f, MathUtils.round(-x, 4, BigDecimal.ROUND_UP), 0.0);
try {
MathUtils.round(1.234f, 2, 1923);
fail();
} catch (IllegalArgumentException ex) {
// success
}
// special values
TestUtils.assertEquals(Float.NaN, MathUtils.round(Float.NaN, 2), 0.0f);
assertEquals(0.0f, MathUtils.round(0.0f, 2), 0.0f);
assertEquals(Float.POSITIVE_INFINITY, MathUtils.round(Float.POSITIVE_INFINITY, 2), 0.0f);
assertEquals(Float.NEGATIVE_INFINITY, MathUtils.round(Float.NEGATIVE_INFINITY, 2), 0.0f);
}
public void testSignByte() {
assertEquals((byte)1, MathUtils.indicator((byte)2));
assertEquals((byte)(-1), MathUtils.indicator((byte)(-2)));
}
public void testSignDouble() {
double delta = 0.0;
assertEquals(1.0, MathUtils.indicator(2.0), delta);
assertEquals(-1.0, MathUtils.indicator(-2.0), delta);
}
public void testSignFloat() {
float delta = 0.0F;
assertEquals(1.0F, MathUtils.indicator(2.0F), delta);
assertEquals(-1.0F, MathUtils.indicator(-2.0F), delta);
}
public void testSignInt() {
assertEquals((int)1, MathUtils.indicator((int)(2)));
assertEquals((int)(-1), MathUtils.indicator((int)(-2)));
}
public void testSignLong() {
assertEquals(1L, MathUtils.indicator(2L));
assertEquals(-1L, MathUtils.indicator(-2L));
}
public void testSignShort() {
assertEquals((short)1, MathUtils.indicator((short)2));
assertEquals((short)(-1), MathUtils.indicator((short)(-2)));
}
public void testSinh() {
double x = 3.0;
double expected = 10.01787;
assertEquals(expected, MathUtils.sinh(x), 1.0e-5);
}
public void testSinhNaN() {
assertTrue(Double.isNaN(MathUtils.sinh(Double.NaN)));
}
public void testSubAndCheck() {
int big = Integer.MAX_VALUE;
int bigNeg = Integer.MIN_VALUE;
assertEquals(big, MathUtils.subAndCheck(big, 0));
try {
MathUtils.subAndCheck(big, -1);
fail("Expecting ArithmeticException");
} catch (ArithmeticException ex) {
}
try {
MathUtils.subAndCheck(bigNeg, 1);
fail("Expecting ArithmeticException");
} catch (ArithmeticException ex) {
}
}
public void testSubAndCheckErrorMessage() {
int big = Integer.MAX_VALUE;
try {
MathUtils.subAndCheck(big, -1);
fail("Expecting ArithmeticException");
} catch (ArithmeticException ex) {
assertEquals("overflow: subtract", ex.getMessage());
}
}
public void testSubAndCheckLong() {
long max = Long.MAX_VALUE;
long min = Long.MIN_VALUE;
assertEquals(max, MathUtils.subAndCheck(max, 0));
assertEquals(min, MathUtils.subAndCheck(min, 0));
assertEquals(-max, MathUtils.subAndCheck(0, max));
testSubAndCheckLongFailure(0L, min);
testSubAndCheckLongFailure(max, -1L);
testSubAndCheckLongFailure(min, 1L);
}
private void testSubAndCheckLongFailure(long a, long b) {
try {
MathUtils.subAndCheck(a, b);
fail("Expecting ArithmeticException");
} catch (ArithmeticException ex) {
// success
}
}
} | // You are a professional Java test case writer, please create a test case named `testGcd` for the issue `Math-MATH-238`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Math-MATH-238
//
// ## Issue-Title:
// MathUtils.gcd(u, v) fails when u and v both contain a high power of 2
//
// ## Issue-Description:
//
// The test at the beginning of MathUtils.gcd(u, v) for arguments equal to zero fails when u and v contain high enough powers of 2 so that their product overflows to zero.
//
//
// assertEquals(3 \* (1<<15), MathUtils.gcd(3 \* (1<<20), 9 \* (1<<15)));
//
//
// Fix: Replace the test at the start of MathUtils.gcd()
//
//
// if (u \* v == 0) {
//
//
// by
//
//
// if (u == 0 || v == 0) {
//
//
//
//
//
public void testGcd() {
| 296 | 94 | 272 | src/test/org/apache/commons/math/util/MathUtilsTest.java | src/test | ```markdown
## Issue-ID: Math-MATH-238
## Issue-Title:
MathUtils.gcd(u, v) fails when u and v both contain a high power of 2
## Issue-Description:
The test at the beginning of MathUtils.gcd(u, v) for arguments equal to zero fails when u and v contain high enough powers of 2 so that their product overflows to zero.
assertEquals(3 \* (1<<15), MathUtils.gcd(3 \* (1<<20), 9 \* (1<<15)));
Fix: Replace the test at the start of MathUtils.gcd()
if (u \* v == 0) {
by
if (u == 0 || v == 0) {
```
You are a professional Java test case writer, please create a test case named `testGcd` for the issue `Math-MATH-238`, utilizing the provided issue report information and the following function signature.
```java
public void testGcd() {
```
| 272 | [
"org.apache.commons.math.util.MathUtils"
] | 9d92b388fdc54b18fa11d6d73c1e73fc4c5e1d65df29af7851335f4a0dbade7e | public void testGcd() | // You are a professional Java test case writer, please create a test case named `testGcd` for the issue `Math-MATH-238`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Math-MATH-238
//
// ## Issue-Title:
// MathUtils.gcd(u, v) fails when u and v both contain a high power of 2
//
// ## Issue-Description:
//
// The test at the beginning of MathUtils.gcd(u, v) for arguments equal to zero fails when u and v contain high enough powers of 2 so that their product overflows to zero.
//
//
// assertEquals(3 \* (1<<15), MathUtils.gcd(3 \* (1<<20), 9 \* (1<<15)));
//
//
// Fix: Replace the test at the start of MathUtils.gcd()
//
//
// if (u \* v == 0) {
//
//
// by
//
//
// if (u == 0 || v == 0) {
//
//
//
//
//
| Math | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with this
* work for additional information regarding copyright ownership. The ASF
* licenses this file to You under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0 Unless required by applicable law
* or agreed to in writing, software distributed under the License is
* distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package org.apache.commons.math.util;
import java.math.BigDecimal;
import junit.framework.Test;
import junit.framework.TestCase;
import junit.framework.TestSuite;
import org.apache.commons.math.random.RandomDataImpl;
import org.apache.commons.math.TestUtils;
/**
* Test cases for the MathUtils class.
* @version $Revision$ $Date: 2007-08-16 15:36:33 -0500 (Thu, 16 Aug
* 2007) $
*/
public final class MathUtilsTest extends TestCase {
public MathUtilsTest(String name) {
super(name);
}
public static Test suite() {
TestSuite suite = new TestSuite(MathUtilsTest.class);
suite.setName("MathUtils Tests");
return suite;
}
/**
* Exact recursive implementation to test against
*/
private long binomialCoefficient(int n, int k) {
if ((n == k) || (k == 0)) {
return 1;
}
if ((k == 1) || (k == n - 1)) {
return n;
}
return binomialCoefficient(n - 1, k - 1) + binomialCoefficient(n - 1, k);
}
/**
* Exact direct multiplication implementation to test against
*/
private long factorial(int n) {
long result = 1;
for (int i = 2; i <= n; i++) {
result *= i;
}
return result;
}
/** Verify that b(0,0) = 1 */
public void test0Choose0() {
assertEquals(MathUtils.binomialCoefficientDouble(0, 0), 1d, 0);
assertEquals(MathUtils.binomialCoefficientLog(0, 0), 0d, 0);
assertEquals(MathUtils.binomialCoefficient(0, 0), 1);
}
public void testAddAndCheck() {
int big = Integer.MAX_VALUE;
int bigNeg = Integer.MIN_VALUE;
assertEquals(big, MathUtils.addAndCheck(big, 0));
try {
MathUtils.addAndCheck(big, 1);
fail("Expecting ArithmeticException");
} catch (ArithmeticException ex) {
}
try {
MathUtils.addAndCheck(bigNeg, -1);
fail("Expecting ArithmeticException");
} catch (ArithmeticException ex) {
}
}
public void testAddAndCheckLong() {
long max = Long.MAX_VALUE;
long min = Long.MIN_VALUE;
assertEquals(max, MathUtils.addAndCheck(max, 0L));
assertEquals(min, MathUtils.addAndCheck(min, 0L));
assertEquals(max, MathUtils.addAndCheck(0L, max));
assertEquals(min, MathUtils.addAndCheck(0L, min));
assertEquals(1, MathUtils.addAndCheck(-1L, 2L));
assertEquals(1, MathUtils.addAndCheck(2L, -1L));
testAddAndCheckLongFailure(max, 1L);
testAddAndCheckLongFailure(min, -1L);
testAddAndCheckLongFailure(1L, max);
testAddAndCheckLongFailure(-1L, min);
}
private void testAddAndCheckLongFailure(long a, long b) {
try {
MathUtils.addAndCheck(a, b);
fail("Expecting ArithmeticException");
} catch (ArithmeticException ex) {
// success
}
}
public void testBinomialCoefficient() {
long[] bcoef5 = {
1,
5,
10,
10,
5,
1 };
long[] bcoef6 = {
1,
6,
15,
20,
15,
6,
1 };
for (int i = 0; i < 6; i++) {
assertEquals("5 choose " + i, bcoef5[i], MathUtils.binomialCoefficient(5, i));
}
for (int i = 0; i < 7; i++) {
assertEquals("6 choose " + i, bcoef6[i], MathUtils.binomialCoefficient(6, i));
}
for (int n = 1; n < 10; n++) {
for (int k = 0; k <= n; k++) {
assertEquals(n + " choose " + k, binomialCoefficient(n, k), MathUtils.binomialCoefficient(n, k));
assertEquals(n + " choose " + k, (double)binomialCoefficient(n, k), MathUtils.binomialCoefficientDouble(n, k), Double.MIN_VALUE);
assertEquals(n + " choose " + k, Math.log((double)binomialCoefficient(n, k)), MathUtils.binomialCoefficientLog(n, k), 10E-12);
}
}
/*
* Takes a long time for recursion to unwind, but succeeds and yields
* exact value = 2,333,606,220
* assertEquals(MathUtils.binomialCoefficient(34,17),
* binomialCoefficient(34,17));
*/
}
public void testBinomialCoefficientFail() {
try {
MathUtils.binomialCoefficient(4, 5);
fail("expecting IllegalArgumentException");
} catch (IllegalArgumentException ex) {
;
}
try {
MathUtils.binomialCoefficientDouble(4, 5);
fail("expecting IllegalArgumentException");
} catch (IllegalArgumentException ex) {
;
}
try {
MathUtils.binomialCoefficientLog(4, 5);
fail("expecting IllegalArgumentException");
} catch (IllegalArgumentException ex) {
;
}
try {
MathUtils.binomialCoefficient(67, 34);
fail("expecting ArithmeticException");
} catch (ArithmeticException ex) {
;
}
double x = MathUtils.binomialCoefficientDouble(1030, 515);
assertTrue("expecting infinite binomial coefficient", Double.isInfinite(x));
}
public void testCosh() {
double x = 3.0;
double expected = 10.06766;
assertEquals(expected, MathUtils.cosh(x), 1.0e-5);
}
public void testCoshNaN() {
assertTrue(Double.isNaN(MathUtils.cosh(Double.NaN)));
}
public void testEquals() {
double[] testArray = {
Double.NaN,
Double.POSITIVE_INFINITY,
Double.NEGATIVE_INFINITY,
1d,
0d };
for (int i = 0; i < testArray.length; i++) {
for (int j = 0; j < testArray.length; j++) {
if (i == j) {
assertTrue(MathUtils.equals(testArray[i], testArray[j]));
assertTrue(MathUtils.equals(testArray[j], testArray[i]));
} else {
assertTrue(!MathUtils.equals(testArray[i], testArray[j]));
assertTrue(!MathUtils.equals(testArray[j], testArray[i]));
}
}
}
}
public void testArrayEquals() {
assertFalse(MathUtils.equals(new double[] { 1d }, null));
assertFalse(MathUtils.equals(null, new double[] { 1d }));
assertTrue(MathUtils.equals((double[]) null, (double[]) null));
assertFalse(MathUtils.equals(new double[] { 1d }, new double[0]));
assertTrue(MathUtils.equals(new double[] { 1d }, new double[] { 1d }));
assertTrue(MathUtils.equals(new double[] {
Double.NaN, Double.POSITIVE_INFINITY,
Double.NEGATIVE_INFINITY, 1d, 0d
}, new double[] {
Double.NaN, Double.POSITIVE_INFINITY,
Double.NEGATIVE_INFINITY, 1d, 0d
}));
assertFalse(MathUtils.equals(new double[] { Double.POSITIVE_INFINITY },
new double[] { Double.NEGATIVE_INFINITY }));
assertFalse(MathUtils.equals(new double[] { 1d },
new double[] { MathUtils.nextAfter(1d, 2d) }));
}
public void testFactorial() {
for (int i = 1; i < 10; i++) {
assertEquals(i + "! ", factorial(i), MathUtils.factorial(i));
assertEquals(i + "! ", (double)factorial(i), MathUtils.factorialDouble(i), Double.MIN_VALUE);
assertEquals(i + "! ", Math.log((double)factorial(i)), MathUtils.factorialLog(i), 10E-12);
}
assertEquals("0", 1, MathUtils.factorial(0));
assertEquals("0", 1.0d, MathUtils.factorialDouble(0), 1E-14);
assertEquals("0", 0.0d, MathUtils.factorialLog(0), 1E-14);
}
public void testFactorialFail() {
try {
MathUtils.factorial(-1);
fail("expecting IllegalArgumentException");
} catch (IllegalArgumentException ex) {
;
}
try {
MathUtils.factorialDouble(-1);
fail("expecting IllegalArgumentException");
} catch (IllegalArgumentException ex) {
;
}
try {
MathUtils.factorialLog(-1);
fail("expecting IllegalArgumentException");
} catch (IllegalArgumentException ex) {
;
}
try {
MathUtils.factorial(21);
fail("expecting ArithmeticException");
} catch (ArithmeticException ex) {
;
}
assertTrue("expecting infinite factorial value", Double.isInfinite(MathUtils.factorialDouble(171)));
}
public void testGcd() {
int a = 30;
int b = 50;
int c = 77;
assertEquals(0, MathUtils.gcd(0, 0));
assertEquals(b, MathUtils.gcd(0, b));
assertEquals(a, MathUtils.gcd(a, 0));
assertEquals(b, MathUtils.gcd(0, -b));
assertEquals(a, MathUtils.gcd(-a, 0));
assertEquals(10, MathUtils.gcd(a, b));
assertEquals(10, MathUtils.gcd(-a, b));
assertEquals(10, MathUtils.gcd(a, -b));
assertEquals(10, MathUtils.gcd(-a, -b));
assertEquals(1, MathUtils.gcd(a, c));
assertEquals(1, MathUtils.gcd(-a, c));
assertEquals(1, MathUtils.gcd(a, -c));
assertEquals(1, MathUtils.gcd(-a, -c));
assertEquals(3 * (1<<15), MathUtils.gcd(3 * (1<<20), 9 * (1<<15)));
}
public void testHash() {
double[] testArray = {
Double.NaN,
Double.POSITIVE_INFINITY,
Double.NEGATIVE_INFINITY,
1d,
0d,
1E-14,
(1 + 1E-14),
Double.MIN_VALUE,
Double.MAX_VALUE };
for (int i = 0; i < testArray.length; i++) {
for (int j = 0; j < testArray.length; j++) {
if (i == j) {
assertEquals(MathUtils.hash(testArray[i]), MathUtils.hash(testArray[j]));
assertEquals(MathUtils.hash(testArray[j]), MathUtils.hash(testArray[i]));
} else {
assertTrue(MathUtils.hash(testArray[i]) != MathUtils.hash(testArray[j]));
assertTrue(MathUtils.hash(testArray[j]) != MathUtils.hash(testArray[i]));
}
}
}
}
public void testArrayHash() {
assertEquals(0, MathUtils.hash((double[]) null));
assertEquals(MathUtils.hash(new double[] {
Double.NaN, Double.POSITIVE_INFINITY,
Double.NEGATIVE_INFINITY, 1d, 0d
}),
MathUtils.hash(new double[] {
Double.NaN, Double.POSITIVE_INFINITY,
Double.NEGATIVE_INFINITY, 1d, 0d
}));
assertFalse(MathUtils.hash(new double[] { 1d }) ==
MathUtils.hash(new double[] { MathUtils.nextAfter(1d, 2d) }));
assertFalse(MathUtils.hash(new double[] { 1d }) ==
MathUtils.hash(new double[] { 1d, 1d }));
}
/**
* Make sure that permuted arrays do not hash to the same value.
*/
public void testPermutedArrayHash() {
double[] original = new double[10];
double[] permuted = new double[10];
RandomDataImpl random = new RandomDataImpl();
// Generate 10 distinct random values
for (int i = 0; i < 10; i++) {
original[i] = random.nextUniform((double)i + 0.5, (double)i + 0.75);
}
// Generate a random permutation, making sure it is not the identity
boolean isIdentity = true;
do {
int[] permutation = random.nextPermutation(10, 10);
for (int i = 0; i < 10; i++) {
if (i != permutation[i]) {
isIdentity = false;
}
permuted[i] = original[permutation[i]];
}
} while (isIdentity);
// Verify that permuted array has different hash
assertFalse(MathUtils.hash(original) == MathUtils.hash(permuted));
}
public void testIndicatorByte() {
assertEquals((byte)1, MathUtils.indicator((byte)2));
assertEquals((byte)1, MathUtils.indicator((byte)0));
assertEquals((byte)(-1), MathUtils.indicator((byte)(-2)));
}
public void testIndicatorDouble() {
double delta = 0.0;
assertEquals(1.0, MathUtils.indicator(2.0), delta);
assertEquals(1.0, MathUtils.indicator(0.0), delta);
assertEquals(-1.0, MathUtils.indicator(-2.0), delta);
}
public void testIndicatorFloat() {
float delta = 0.0F;
assertEquals(1.0F, MathUtils.indicator(2.0F), delta);
assertEquals(1.0F, MathUtils.indicator(0.0F), delta);
assertEquals(-1.0F, MathUtils.indicator(-2.0F), delta);
}
public void testIndicatorInt() {
assertEquals((int)1, MathUtils.indicator((int)(2)));
assertEquals((int)1, MathUtils.indicator((int)(0)));
assertEquals((int)(-1), MathUtils.indicator((int)(-2)));
}
public void testIndicatorLong() {
assertEquals(1L, MathUtils.indicator(2L));
assertEquals(1L, MathUtils.indicator(0L));
assertEquals(-1L, MathUtils.indicator(-2L));
}
public void testIndicatorShort() {
assertEquals((short)1, MathUtils.indicator((short)2));
assertEquals((short)1, MathUtils.indicator((short)0));
assertEquals((short)(-1), MathUtils.indicator((short)(-2)));
}
public void testLcm() {
int a = 30;
int b = 50;
int c = 77;
assertEquals(0, MathUtils.lcm(0, b));
assertEquals(0, MathUtils.lcm(a, 0));
assertEquals(b, MathUtils.lcm(1, b));
assertEquals(a, MathUtils.lcm(a, 1));
assertEquals(150, MathUtils.lcm(a, b));
assertEquals(150, MathUtils.lcm(-a, b));
assertEquals(150, MathUtils.lcm(a, -b));
assertEquals(2310, MathUtils.lcm(a, c));
try {
MathUtils.lcm(Integer.MAX_VALUE, Integer.MAX_VALUE - 1);
fail("Expecting ArithmeticException");
} catch (ArithmeticException ex) {
// expected
}
}
public void testLog() {
assertEquals(2.0, MathUtils.log(2, 4), 0);
assertEquals(3.0, MathUtils.log(2, 8), 0);
assertTrue(Double.isNaN(MathUtils.log(-1, 1)));
assertTrue(Double.isNaN(MathUtils.log(1, -1)));
assertTrue(Double.isNaN(MathUtils.log(0, 0)));
assertEquals(0, MathUtils.log(0, 10), 0);
assertEquals(Double.NEGATIVE_INFINITY, MathUtils.log(10, 0), 0);
}
public void testMulAndCheck() {
int big = Integer.MAX_VALUE;
int bigNeg = Integer.MIN_VALUE;
assertEquals(big, MathUtils.mulAndCheck(big, 1));
try {
MathUtils.mulAndCheck(big, 2);
fail("Expecting ArithmeticException");
} catch (ArithmeticException ex) {
}
try {
MathUtils.mulAndCheck(bigNeg, 2);
fail("Expecting ArithmeticException");
} catch (ArithmeticException ex) {
}
}
public void testMulAndCheckLong() {
long max = Long.MAX_VALUE;
long min = Long.MIN_VALUE;
assertEquals(max, MathUtils.mulAndCheck(max, 1L));
assertEquals(min, MathUtils.mulAndCheck(min, 1L));
assertEquals(0L, MathUtils.mulAndCheck(max, 0L));
assertEquals(0L, MathUtils.mulAndCheck(min, 0L));
assertEquals(max, MathUtils.mulAndCheck(1L, max));
assertEquals(min, MathUtils.mulAndCheck(1L, min));
assertEquals(0L, MathUtils.mulAndCheck(0L, max));
assertEquals(0L, MathUtils.mulAndCheck(0L, min));
testMulAndCheckLongFailure(max, 2L);
testMulAndCheckLongFailure(2L, max);
testMulAndCheckLongFailure(min, 2L);
testMulAndCheckLongFailure(2L, min);
testMulAndCheckLongFailure(min, -1L);
testMulAndCheckLongFailure(-1L, min);
}
private void testMulAndCheckLongFailure(long a, long b) {
try {
MathUtils.mulAndCheck(a, b);
fail("Expecting ArithmeticException");
} catch (ArithmeticException ex) {
// success
}
}
public void testNextAfter() {
// 0x402fffffffffffff 0x404123456789abcd -> 4030000000000000
assertEquals(16.0, MathUtils.nextAfter(15.999999999999998, 34.27555555555555), 0.0);
// 0xc02fffffffffffff 0x404123456789abcd -> c02ffffffffffffe
assertEquals(-15.999999999999996, MathUtils.nextAfter(-15.999999999999998, 34.27555555555555), 0.0);
// 0x402fffffffffffff 0x400123456789abcd -> 402ffffffffffffe
assertEquals(15.999999999999996, MathUtils.nextAfter(15.999999999999998, 2.142222222222222), 0.0);
// 0xc02fffffffffffff 0x400123456789abcd -> c02ffffffffffffe
assertEquals(-15.999999999999996, MathUtils.nextAfter(-15.999999999999998, 2.142222222222222), 0.0);
// 0x4020000000000000 0x404123456789abcd -> 4020000000000001
assertEquals(8.000000000000002, MathUtils.nextAfter(8.0, 34.27555555555555), 0.0);
// 0xc020000000000000 0x404123456789abcd -> c01fffffffffffff
assertEquals(-7.999999999999999, MathUtils.nextAfter(-8.0, 34.27555555555555), 0.0);
// 0x4020000000000000 0x400123456789abcd -> 401fffffffffffff
assertEquals(7.999999999999999, MathUtils.nextAfter(8.0, 2.142222222222222), 0.0);
// 0xc020000000000000 0x400123456789abcd -> c01fffffffffffff
assertEquals(-7.999999999999999, MathUtils.nextAfter(-8.0, 2.142222222222222), 0.0);
// 0x3f2e43753d36a223 0x3f2e43753d36a224 -> 3f2e43753d36a224
assertEquals(2.308922399667661E-4, MathUtils.nextAfter(2.3089223996676606E-4, 2.308922399667661E-4), 0.0);
// 0x3f2e43753d36a223 0x3f2e43753d36a223 -> 3f2e43753d36a224
assertEquals(2.308922399667661E-4, MathUtils.nextAfter(2.3089223996676606E-4, 2.3089223996676606E-4), 0.0);
// 0x3f2e43753d36a223 0x3f2e43753d36a222 -> 3f2e43753d36a222
assertEquals(2.3089223996676603E-4, MathUtils.nextAfter(2.3089223996676606E-4, 2.3089223996676603E-4), 0.0);
// 0x3f2e43753d36a223 0xbf2e43753d36a224 -> 3f2e43753d36a222
assertEquals(2.3089223996676603E-4, MathUtils.nextAfter(2.3089223996676606E-4, -2.308922399667661E-4), 0.0);
// 0x3f2e43753d36a223 0xbf2e43753d36a223 -> 3f2e43753d36a222
assertEquals(2.3089223996676603E-4, MathUtils.nextAfter(2.3089223996676606E-4, -2.3089223996676606E-4), 0.0);
// 0x3f2e43753d36a223 0xbf2e43753d36a222 -> 3f2e43753d36a222
assertEquals(2.3089223996676603E-4, MathUtils.nextAfter(2.3089223996676606E-4, -2.3089223996676603E-4), 0.0);
// 0xbf2e43753d36a223 0x3f2e43753d36a224 -> bf2e43753d36a222
assertEquals(-2.3089223996676603E-4, MathUtils.nextAfter(-2.3089223996676606E-4, 2.308922399667661E-4), 0.0);
// 0xbf2e43753d36a223 0x3f2e43753d36a223 -> bf2e43753d36a222
assertEquals(-2.3089223996676603E-4, MathUtils.nextAfter(-2.3089223996676606E-4, 2.3089223996676606E-4), 0.0);
// 0xbf2e43753d36a223 0x3f2e43753d36a222 -> bf2e43753d36a222
assertEquals(-2.3089223996676603E-4, MathUtils.nextAfter(-2.3089223996676606E-4, 2.3089223996676603E-4), 0.0);
// 0xbf2e43753d36a223 0xbf2e43753d36a224 -> bf2e43753d36a224
assertEquals(-2.308922399667661E-4, MathUtils.nextAfter(-2.3089223996676606E-4, -2.308922399667661E-4), 0.0);
// 0xbf2e43753d36a223 0xbf2e43753d36a223 -> bf2e43753d36a224
assertEquals(-2.308922399667661E-4, MathUtils.nextAfter(-2.3089223996676606E-4, -2.3089223996676606E-4), 0.0);
// 0xbf2e43753d36a223 0xbf2e43753d36a222 -> bf2e43753d36a222
assertEquals(-2.3089223996676603E-4, MathUtils.nextAfter(-2.3089223996676606E-4, -2.3089223996676603E-4), 0.0);
}
public void testNextAfterSpecialCases() {
assertTrue(Double.isInfinite(MathUtils.nextAfter(Double.NEGATIVE_INFINITY, 0)));
assertTrue(Double.isInfinite(MathUtils.nextAfter(Double.POSITIVE_INFINITY, 0)));
assertTrue(Double.isNaN(MathUtils.nextAfter(Double.NaN, 0)));
assertTrue(Double.isInfinite(MathUtils.nextAfter(Double.MAX_VALUE, Double.POSITIVE_INFINITY)));
assertTrue(Double.isInfinite(MathUtils.nextAfter(-Double.MAX_VALUE, Double.NEGATIVE_INFINITY)));
assertEquals(Double.MIN_VALUE, MathUtils.nextAfter(0, 1), 0);
assertEquals(-Double.MIN_VALUE, MathUtils.nextAfter(0, -1), 0);
assertEquals(0, MathUtils.nextAfter(Double.MIN_VALUE, -1), 0);
assertEquals(0, MathUtils.nextAfter(-Double.MIN_VALUE, 1), 0);
}
public void testScalb() {
assertEquals( 0.0, MathUtils.scalb(0.0, 5), 1.0e-15);
assertEquals(32.0, MathUtils.scalb(1.0, 5), 1.0e-15);
assertEquals(1.0 / 32.0, MathUtils.scalb(1.0, -5), 1.0e-15);
assertEquals(Math.PI, MathUtils.scalb(Math.PI, 0), 1.0e-15);
assertTrue(Double.isInfinite(MathUtils.scalb(Double.POSITIVE_INFINITY, 1)));
assertTrue(Double.isInfinite(MathUtils.scalb(Double.NEGATIVE_INFINITY, 1)));
assertTrue(Double.isNaN(MathUtils.scalb(Double.NaN, 1)));
}
public void testNormalizeAngle() {
for (double a = -15.0; a <= 15.0; a += 0.1) {
for (double b = -15.0; b <= 15.0; b += 0.2) {
double c = MathUtils.normalizeAngle(a, b);
assertTrue((b - Math.PI) <= c);
assertTrue(c <= (b + Math.PI));
double twoK = Math.rint((a - c) / Math.PI);
assertEquals(c, a - twoK * Math.PI, 1.0e-14);
}
}
}
public void testRoundDouble() {
double x = 1.234567890;
assertEquals(1.23, MathUtils.round(x, 2), 0.0);
assertEquals(1.235, MathUtils.round(x, 3), 0.0);
assertEquals(1.2346, MathUtils.round(x, 4), 0.0);
// JIRA MATH-151
assertEquals(39.25, MathUtils.round(39.245, 2), 0.0);
assertEquals(39.24, MathUtils.round(39.245, 2, BigDecimal.ROUND_DOWN), 0.0);
double xx = 39.0;
xx = xx + 245d / 1000d;
assertEquals(39.25, MathUtils.round(xx, 2), 0.0);
// BZ 35904
assertEquals(30.1d, MathUtils.round(30.095d, 2), 0.0d);
assertEquals(30.1d, MathUtils.round(30.095d, 1), 0.0d);
assertEquals(33.1d, MathUtils.round(33.095d, 1), 0.0d);
assertEquals(33.1d, MathUtils.round(33.095d, 2), 0.0d);
assertEquals(50.09d, MathUtils.round(50.085d, 2), 0.0d);
assertEquals(50.19d, MathUtils.round(50.185d, 2), 0.0d);
assertEquals(50.01d, MathUtils.round(50.005d, 2), 0.0d);
assertEquals(30.01d, MathUtils.round(30.005d, 2), 0.0d);
assertEquals(30.65d, MathUtils.round(30.645d, 2), 0.0d);
assertEquals(1.24, MathUtils.round(x, 2, BigDecimal.ROUND_CEILING), 0.0);
assertEquals(1.235, MathUtils.round(x, 3, BigDecimal.ROUND_CEILING), 0.0);
assertEquals(1.2346, MathUtils.round(x, 4, BigDecimal.ROUND_CEILING), 0.0);
assertEquals(-1.23, MathUtils.round(-x, 2, BigDecimal.ROUND_CEILING), 0.0);
assertEquals(-1.234, MathUtils.round(-x, 3, BigDecimal.ROUND_CEILING), 0.0);
assertEquals(-1.2345, MathUtils.round(-x, 4, BigDecimal.ROUND_CEILING), 0.0);
assertEquals(1.23, MathUtils.round(x, 2, BigDecimal.ROUND_DOWN), 0.0);
assertEquals(1.234, MathUtils.round(x, 3, BigDecimal.ROUND_DOWN), 0.0);
assertEquals(1.2345, MathUtils.round(x, 4, BigDecimal.ROUND_DOWN), 0.0);
assertEquals(-1.23, MathUtils.round(-x, 2, BigDecimal.ROUND_DOWN), 0.0);
assertEquals(-1.234, MathUtils.round(-x, 3, BigDecimal.ROUND_DOWN), 0.0);
assertEquals(-1.2345, MathUtils.round(-x, 4, BigDecimal.ROUND_DOWN), 0.0);
assertEquals(1.23, MathUtils.round(x, 2, BigDecimal.ROUND_FLOOR), 0.0);
assertEquals(1.234, MathUtils.round(x, 3, BigDecimal.ROUND_FLOOR), 0.0);
assertEquals(1.2345, MathUtils.round(x, 4, BigDecimal.ROUND_FLOOR), 0.0);
assertEquals(-1.24, MathUtils.round(-x, 2, BigDecimal.ROUND_FLOOR), 0.0);
assertEquals(-1.235, MathUtils.round(-x, 3, BigDecimal.ROUND_FLOOR), 0.0);
assertEquals(-1.2346, MathUtils.round(-x, 4, BigDecimal.ROUND_FLOOR), 0.0);
assertEquals(1.23, MathUtils.round(x, 2, BigDecimal.ROUND_HALF_DOWN), 0.0);
assertEquals(1.235, MathUtils.round(x, 3, BigDecimal.ROUND_HALF_DOWN), 0.0);
assertEquals(1.2346, MathUtils.round(x, 4, BigDecimal.ROUND_HALF_DOWN), 0.0);
assertEquals(-1.23, MathUtils.round(-x, 2, BigDecimal.ROUND_HALF_DOWN), 0.0);
assertEquals(-1.235, MathUtils.round(-x, 3, BigDecimal.ROUND_HALF_DOWN), 0.0);
assertEquals(-1.2346, MathUtils.round(-x, 4, BigDecimal.ROUND_HALF_DOWN), 0.0);
assertEquals(1.234, MathUtils.round(1.2345, 3, BigDecimal.ROUND_HALF_DOWN), 0.0);
assertEquals(-1.234, MathUtils.round(-1.2345, 3, BigDecimal.ROUND_HALF_DOWN), 0.0);
assertEquals(1.23, MathUtils.round(x, 2, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(1.235, MathUtils.round(x, 3, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(1.2346, MathUtils.round(x, 4, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(-1.23, MathUtils.round(-x, 2, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(-1.235, MathUtils.round(-x, 3, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(-1.2346, MathUtils.round(-x, 4, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(1.234, MathUtils.round(1.2345, 3, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(-1.234, MathUtils.round(-1.2345, 3, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(1.236, MathUtils.round(1.2355, 3, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(-1.236, MathUtils.round(-1.2355, 3, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(1.23, MathUtils.round(x, 2, BigDecimal.ROUND_HALF_UP), 0.0);
assertEquals(1.235, MathUtils.round(x, 3, BigDecimal.ROUND_HALF_UP), 0.0);
assertEquals(1.2346, MathUtils.round(x, 4, BigDecimal.ROUND_HALF_UP), 0.0);
assertEquals(-1.23, MathUtils.round(-x, 2, BigDecimal.ROUND_HALF_UP), 0.0);
assertEquals(-1.235, MathUtils.round(-x, 3, BigDecimal.ROUND_HALF_UP), 0.0);
assertEquals(-1.2346, MathUtils.round(-x, 4, BigDecimal.ROUND_HALF_UP), 0.0);
assertEquals(1.235, MathUtils.round(1.2345, 3, BigDecimal.ROUND_HALF_UP), 0.0);
assertEquals(-1.235, MathUtils.round(-1.2345, 3, BigDecimal.ROUND_HALF_UP), 0.0);
assertEquals(-1.23, MathUtils.round(-1.23, 2, BigDecimal.ROUND_UNNECESSARY), 0.0);
assertEquals(1.23, MathUtils.round(1.23, 2, BigDecimal.ROUND_UNNECESSARY), 0.0);
try {
MathUtils.round(1.234, 2, BigDecimal.ROUND_UNNECESSARY);
fail();
} catch (ArithmeticException ex) {
// success
}
assertEquals(1.24, MathUtils.round(x, 2, BigDecimal.ROUND_UP), 0.0);
assertEquals(1.235, MathUtils.round(x, 3, BigDecimal.ROUND_UP), 0.0);
assertEquals(1.2346, MathUtils.round(x, 4, BigDecimal.ROUND_UP), 0.0);
assertEquals(-1.24, MathUtils.round(-x, 2, BigDecimal.ROUND_UP), 0.0);
assertEquals(-1.235, MathUtils.round(-x, 3, BigDecimal.ROUND_UP), 0.0);
assertEquals(-1.2346, MathUtils.round(-x, 4, BigDecimal.ROUND_UP), 0.0);
try {
MathUtils.round(1.234, 2, 1923);
fail();
} catch (IllegalArgumentException ex) {
// success
}
// MATH-151
assertEquals(39.25, MathUtils.round(39.245, 2, BigDecimal.ROUND_HALF_UP), 0.0);
// special values
TestUtils.assertEquals(Double.NaN, MathUtils.round(Double.NaN, 2), 0.0);
assertEquals(0.0, MathUtils.round(0.0, 2), 0.0);
assertEquals(Double.POSITIVE_INFINITY, MathUtils.round(Double.POSITIVE_INFINITY, 2), 0.0);
assertEquals(Double.NEGATIVE_INFINITY, MathUtils.round(Double.NEGATIVE_INFINITY, 2), 0.0);
}
public void testRoundFloat() {
float x = 1.234567890f;
assertEquals(1.23f, MathUtils.round(x, 2), 0.0);
assertEquals(1.235f, MathUtils.round(x, 3), 0.0);
assertEquals(1.2346f, MathUtils.round(x, 4), 0.0);
// BZ 35904
assertEquals(30.1f, MathUtils.round(30.095f, 2), 0.0f);
assertEquals(30.1f, MathUtils.round(30.095f, 1), 0.0f);
assertEquals(50.09f, MathUtils.round(50.085f, 2), 0.0f);
assertEquals(50.19f, MathUtils.round(50.185f, 2), 0.0f);
assertEquals(50.01f, MathUtils.round(50.005f, 2), 0.0f);
assertEquals(30.01f, MathUtils.round(30.005f, 2), 0.0f);
assertEquals(30.65f, MathUtils.round(30.645f, 2), 0.0f);
assertEquals(1.24f, MathUtils.round(x, 2, BigDecimal.ROUND_CEILING), 0.0);
assertEquals(1.235f, MathUtils.round(x, 3, BigDecimal.ROUND_CEILING), 0.0);
assertEquals(1.2346f, MathUtils.round(x, 4, BigDecimal.ROUND_CEILING), 0.0);
assertEquals(-1.23f, MathUtils.round(-x, 2, BigDecimal.ROUND_CEILING), 0.0);
assertEquals(-1.234f, MathUtils.round(-x, 3, BigDecimal.ROUND_CEILING), 0.0);
assertEquals(-1.2345f, MathUtils.round(-x, 4, BigDecimal.ROUND_CEILING), 0.0);
assertEquals(1.23f, MathUtils.round(x, 2, BigDecimal.ROUND_DOWN), 0.0);
assertEquals(1.234f, MathUtils.round(x, 3, BigDecimal.ROUND_DOWN), 0.0);
assertEquals(1.2345f, MathUtils.round(x, 4, BigDecimal.ROUND_DOWN), 0.0);
assertEquals(-1.23f, MathUtils.round(-x, 2, BigDecimal.ROUND_DOWN), 0.0);
assertEquals(-1.234f, MathUtils.round(-x, 3, BigDecimal.ROUND_DOWN), 0.0);
assertEquals(-1.2345f, MathUtils.round(-x, 4, BigDecimal.ROUND_DOWN), 0.0);
assertEquals(1.23f, MathUtils.round(x, 2, BigDecimal.ROUND_FLOOR), 0.0);
assertEquals(1.234f, MathUtils.round(x, 3, BigDecimal.ROUND_FLOOR), 0.0);
assertEquals(1.2345f, MathUtils.round(x, 4, BigDecimal.ROUND_FLOOR), 0.0);
assertEquals(-1.24f, MathUtils.round(-x, 2, BigDecimal.ROUND_FLOOR), 0.0);
assertEquals(-1.235f, MathUtils.round(-x, 3, BigDecimal.ROUND_FLOOR), 0.0);
assertEquals(-1.2346f, MathUtils.round(-x, 4, BigDecimal.ROUND_FLOOR), 0.0);
assertEquals(1.23f, MathUtils.round(x, 2, BigDecimal.ROUND_HALF_DOWN), 0.0);
assertEquals(1.235f, MathUtils.round(x, 3, BigDecimal.ROUND_HALF_DOWN), 0.0);
assertEquals(1.2346f, MathUtils.round(x, 4, BigDecimal.ROUND_HALF_DOWN), 0.0);
assertEquals(-1.23f, MathUtils.round(-x, 2, BigDecimal.ROUND_HALF_DOWN), 0.0);
assertEquals(-1.235f, MathUtils.round(-x, 3, BigDecimal.ROUND_HALF_DOWN), 0.0);
assertEquals(-1.2346f, MathUtils.round(-x, 4, BigDecimal.ROUND_HALF_DOWN), 0.0);
assertEquals(1.234f, MathUtils.round(1.2345f, 3, BigDecimal.ROUND_HALF_DOWN), 0.0);
assertEquals(-1.234f, MathUtils.round(-1.2345f, 3, BigDecimal.ROUND_HALF_DOWN), 0.0);
assertEquals(1.23f, MathUtils.round(x, 2, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(1.235f, MathUtils.round(x, 3, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(1.2346f, MathUtils.round(x, 4, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(-1.23f, MathUtils.round(-x, 2, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(-1.235f, MathUtils.round(-x, 3, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(-1.2346f, MathUtils.round(-x, 4, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(1.234f, MathUtils.round(1.2345f, 3, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(-1.234f, MathUtils.round(-1.2345f, 3, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(1.236f, MathUtils.round(1.2355f, 3, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(-1.236f, MathUtils.round(-1.2355f, 3, BigDecimal.ROUND_HALF_EVEN), 0.0);
assertEquals(1.23f, MathUtils.round(x, 2, BigDecimal.ROUND_HALF_UP), 0.0);
assertEquals(1.235f, MathUtils.round(x, 3, BigDecimal.ROUND_HALF_UP), 0.0);
assertEquals(1.2346f, MathUtils.round(x, 4, BigDecimal.ROUND_HALF_UP), 0.0);
assertEquals(-1.23f, MathUtils.round(-x, 2, BigDecimal.ROUND_HALF_UP), 0.0);
assertEquals(-1.235f, MathUtils.round(-x, 3, BigDecimal.ROUND_HALF_UP), 0.0);
assertEquals(-1.2346f, MathUtils.round(-x, 4, BigDecimal.ROUND_HALF_UP), 0.0);
assertEquals(1.235f, MathUtils.round(1.2345f, 3, BigDecimal.ROUND_HALF_UP), 0.0);
assertEquals(-1.235f, MathUtils.round(-1.2345f, 3, BigDecimal.ROUND_HALF_UP), 0.0);
assertEquals(-1.23f, MathUtils.round(-1.23f, 2, BigDecimal.ROUND_UNNECESSARY), 0.0);
assertEquals(1.23f, MathUtils.round(1.23f, 2, BigDecimal.ROUND_UNNECESSARY), 0.0);
try {
MathUtils.round(1.234f, 2, BigDecimal.ROUND_UNNECESSARY);
fail();
} catch (ArithmeticException ex) {
// success
}
assertEquals(1.24f, MathUtils.round(x, 2, BigDecimal.ROUND_UP), 0.0);
assertEquals(1.235f, MathUtils.round(x, 3, BigDecimal.ROUND_UP), 0.0);
assertEquals(1.2346f, MathUtils.round(x, 4, BigDecimal.ROUND_UP), 0.0);
assertEquals(-1.24f, MathUtils.round(-x, 2, BigDecimal.ROUND_UP), 0.0);
assertEquals(-1.235f, MathUtils.round(-x, 3, BigDecimal.ROUND_UP), 0.0);
assertEquals(-1.2346f, MathUtils.round(-x, 4, BigDecimal.ROUND_UP), 0.0);
try {
MathUtils.round(1.234f, 2, 1923);
fail();
} catch (IllegalArgumentException ex) {
// success
}
// special values
TestUtils.assertEquals(Float.NaN, MathUtils.round(Float.NaN, 2), 0.0f);
assertEquals(0.0f, MathUtils.round(0.0f, 2), 0.0f);
assertEquals(Float.POSITIVE_INFINITY, MathUtils.round(Float.POSITIVE_INFINITY, 2), 0.0f);
assertEquals(Float.NEGATIVE_INFINITY, MathUtils.round(Float.NEGATIVE_INFINITY, 2), 0.0f);
}
public void testSignByte() {
assertEquals((byte)1, MathUtils.indicator((byte)2));
assertEquals((byte)(-1), MathUtils.indicator((byte)(-2)));
}
public void testSignDouble() {
double delta = 0.0;
assertEquals(1.0, MathUtils.indicator(2.0), delta);
assertEquals(-1.0, MathUtils.indicator(-2.0), delta);
}
public void testSignFloat() {
float delta = 0.0F;
assertEquals(1.0F, MathUtils.indicator(2.0F), delta);
assertEquals(-1.0F, MathUtils.indicator(-2.0F), delta);
}
public void testSignInt() {
assertEquals((int)1, MathUtils.indicator((int)(2)));
assertEquals((int)(-1), MathUtils.indicator((int)(-2)));
}
public void testSignLong() {
assertEquals(1L, MathUtils.indicator(2L));
assertEquals(-1L, MathUtils.indicator(-2L));
}
public void testSignShort() {
assertEquals((short)1, MathUtils.indicator((short)2));
assertEquals((short)(-1), MathUtils.indicator((short)(-2)));
}
public void testSinh() {
double x = 3.0;
double expected = 10.01787;
assertEquals(expected, MathUtils.sinh(x), 1.0e-5);
}
public void testSinhNaN() {
assertTrue(Double.isNaN(MathUtils.sinh(Double.NaN)));
}
public void testSubAndCheck() {
int big = Integer.MAX_VALUE;
int bigNeg = Integer.MIN_VALUE;
assertEquals(big, MathUtils.subAndCheck(big, 0));
try {
MathUtils.subAndCheck(big, -1);
fail("Expecting ArithmeticException");
} catch (ArithmeticException ex) {
}
try {
MathUtils.subAndCheck(bigNeg, 1);
fail("Expecting ArithmeticException");
} catch (ArithmeticException ex) {
}
}
public void testSubAndCheckErrorMessage() {
int big = Integer.MAX_VALUE;
try {
MathUtils.subAndCheck(big, -1);
fail("Expecting ArithmeticException");
} catch (ArithmeticException ex) {
assertEquals("overflow: subtract", ex.getMessage());
}
}
public void testSubAndCheckLong() {
long max = Long.MAX_VALUE;
long min = Long.MIN_VALUE;
assertEquals(max, MathUtils.subAndCheck(max, 0));
assertEquals(min, MathUtils.subAndCheck(min, 0));
assertEquals(-max, MathUtils.subAndCheck(0, max));
testSubAndCheckLongFailure(0L, min);
testSubAndCheckLongFailure(max, -1L);
testSubAndCheckLongFailure(min, 1L);
}
private void testSubAndCheckLongFailure(long a, long b) {
try {
MathUtils.subAndCheck(a, b);
fail("Expecting ArithmeticException");
} catch (ArithmeticException ex) {
// success
}
}
} |
||
@Test
public void testHashAndEquals() {
String doc1 = "<div id=1><p class=one>One</p><p class=one>One</p><p class=one>Two</p><p class=two>One</p></div>" +
"<div id=2><p class=one>One</p><p class=one>One</p><p class=one>Two</p><p class=two>One</p></div>";
Document doc = Jsoup.parse(doc1);
Elements els = doc.select("p");
/*
for (Element el : els) {
System.out.println(el.hashCode() + " - " + el.outerHtml());
}
0 1534787905 - <p class="one">One</p>
1 1534787905 - <p class="one">One</p>
2 1539683239 - <p class="one">Two</p>
3 1535455211 - <p class="two">One</p>
4 1534787905 - <p class="one">One</p>
5 1534787905 - <p class="one">One</p>
6 1539683239 - <p class="one">Two</p>
7 1535455211 - <p class="two">One</p>
*/
assertEquals(8, els.size());
Element e0 = els.get(0);
Element e1 = els.get(1);
Element e2 = els.get(2);
Element e3 = els.get(3);
Element e4 = els.get(4);
Element e5 = els.get(5);
Element e6 = els.get(6);
Element e7 = els.get(7);
assertEquals(e0, e1);
assertEquals(e0, e4);
assertEquals(e0, e5);
assertFalse(e0.equals(e2));
assertFalse(e0.equals(e3));
assertFalse(e0.equals(e6));
assertFalse(e0.equals(e7));
assertEquals(e0.hashCode(), e1.hashCode());
assertEquals(e0.hashCode(), e4.hashCode());
assertEquals(e0.hashCode(), e5.hashCode());
assertFalse(e0.hashCode() == (e2.hashCode()));
assertFalse(e0.hashCode() == (e3).hashCode());
assertFalse(e0.hashCode() == (e6).hashCode());
assertFalse(e0.hashCode() == (e7).hashCode());
} | org.jsoup.nodes.ElementTest::testHashAndEquals | src/test/java/org/jsoup/nodes/ElementTest.java | 799 | src/test/java/org/jsoup/nodes/ElementTest.java | testHashAndEquals | package org.jsoup.nodes;
import org.jsoup.Jsoup;
import org.jsoup.TextUtil;
import org.jsoup.helper.StringUtil;
import org.jsoup.parser.Tag;
import org.jsoup.select.Elements;
import org.junit.Test;
import static org.junit.Assert.*;
import java.util.ArrayList;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Set;
import java.util.Map;
/**
* Tests for Element (DOM stuff mostly).
*
* @author Jonathan Hedley
*/
public class ElementTest {
private String reference = "<div id=div1><p>Hello</p><p>Another <b>element</b></p><div id=div2><img src=foo.png></div></div>";
@Test public void getElementsByTagName() {
Document doc = Jsoup.parse(reference);
List<Element> divs = doc.getElementsByTag("div");
assertEquals(2, divs.size());
assertEquals("div1", divs.get(0).id());
assertEquals("div2", divs.get(1).id());
List<Element> ps = doc.getElementsByTag("p");
assertEquals(2, ps.size());
assertEquals("Hello", ((TextNode) ps.get(0).childNode(0)).getWholeText());
assertEquals("Another ", ((TextNode) ps.get(1).childNode(0)).getWholeText());
List<Element> ps2 = doc.getElementsByTag("P");
assertEquals(ps, ps2);
List<Element> imgs = doc.getElementsByTag("img");
assertEquals("foo.png", imgs.get(0).attr("src"));
List<Element> empty = doc.getElementsByTag("wtf");
assertEquals(0, empty.size());
}
@Test public void getNamespacedElementsByTag() {
Document doc = Jsoup.parse("<div><abc:def id=1>Hello</abc:def></div>");
Elements els = doc.getElementsByTag("abc:def");
assertEquals(1, els.size());
assertEquals("1", els.first().id());
assertEquals("abc:def", els.first().tagName());
}
@Test public void testGetElementById() {
Document doc = Jsoup.parse(reference);
Element div = doc.getElementById("div1");
assertEquals("div1", div.id());
assertNull(doc.getElementById("none"));
Document doc2 = Jsoup.parse("<div id=1><div id=2><p>Hello <span id=2>world!</span></p></div></div>");
Element div2 = doc2.getElementById("2");
assertEquals("div", div2.tagName()); // not the span
Element span = div2.child(0).getElementById("2"); // called from <p> context should be span
assertEquals("span", span.tagName());
}
@Test public void testGetText() {
Document doc = Jsoup.parse(reference);
assertEquals("Hello Another element", doc.text());
assertEquals("Another element", doc.getElementsByTag("p").get(1).text());
}
@Test public void testGetChildText() {
Document doc = Jsoup.parse("<p>Hello <b>there</b> now");
Element p = doc.select("p").first();
assertEquals("Hello there now", p.text());
assertEquals("Hello now", p.ownText());
}
@Test public void testNormalisesText() {
String h = "<p>Hello<p>There.</p> \n <p>Here <b>is</b> \n s<b>om</b>e text.";
Document doc = Jsoup.parse(h);
String text = doc.text();
assertEquals("Hello There. Here is some text.", text);
}
@Test public void testKeepsPreText() {
String h = "<p>Hello \n \n there.</p> <div><pre> What's \n\n that?</pre>";
Document doc = Jsoup.parse(h);
assertEquals("Hello there. What's \n\n that?", doc.text());
}
@Test public void testKeepsPreTextInCode() {
String h = "<pre><code>code\n\ncode</code></pre>";
Document doc = Jsoup.parse(h);
assertEquals("code\n\ncode", doc.text());
assertEquals("<pre><code>code\n\ncode</code></pre>", doc.body().html());
}
@Test public void testBrHasSpace() {
Document doc = Jsoup.parse("<p>Hello<br>there</p>");
assertEquals("Hello there", doc.text());
assertEquals("Hello there", doc.select("p").first().ownText());
doc = Jsoup.parse("<p>Hello <br> there</p>");
assertEquals("Hello there", doc.text());
}
@Test public void testGetSiblings() {
Document doc = Jsoup.parse("<div><p>Hello<p id=1>there<p>this<p>is<p>an<p id=last>element</div>");
Element p = doc.getElementById("1");
assertEquals("there", p.text());
assertEquals("Hello", p.previousElementSibling().text());
assertEquals("this", p.nextElementSibling().text());
assertEquals("Hello", p.firstElementSibling().text());
assertEquals("element", p.lastElementSibling().text());
}
@Test public void testGetParents() {
Document doc = Jsoup.parse("<div><p>Hello <span>there</span></div>");
Element span = doc.select("span").first();
Elements parents = span.parents();
assertEquals(4, parents.size());
assertEquals("p", parents.get(0).tagName());
assertEquals("div", parents.get(1).tagName());
assertEquals("body", parents.get(2).tagName());
assertEquals("html", parents.get(3).tagName());
}
@Test public void testElementSiblingIndex() {
Document doc = Jsoup.parse("<div><p>One</p>...<p>Two</p>...<p>Three</p>");
Elements ps = doc.select("p");
assertTrue(0 == ps.get(0).elementSiblingIndex());
assertTrue(1 == ps.get(1).elementSiblingIndex());
assertTrue(2 == ps.get(2).elementSiblingIndex());
}
@Test public void testGetElementsWithClass() {
Document doc = Jsoup.parse("<div class='mellow yellow'><span class=mellow>Hello <b class='yellow'>Yellow!</b></span><p>Empty</p></div>");
List<Element> els = doc.getElementsByClass("mellow");
assertEquals(2, els.size());
assertEquals("div", els.get(0).tagName());
assertEquals("span", els.get(1).tagName());
List<Element> els2 = doc.getElementsByClass("yellow");
assertEquals(2, els2.size());
assertEquals("div", els2.get(0).tagName());
assertEquals("b", els2.get(1).tagName());
List<Element> none = doc.getElementsByClass("solo");
assertEquals(0, none.size());
}
@Test public void testGetElementsWithAttribute() {
Document doc = Jsoup.parse("<div style='bold'><p title=qux><p><b style></b></p></div>");
List<Element> els = doc.getElementsByAttribute("style");
assertEquals(2, els.size());
assertEquals("div", els.get(0).tagName());
assertEquals("b", els.get(1).tagName());
List<Element> none = doc.getElementsByAttribute("class");
assertEquals(0, none.size());
}
@Test public void testGetElementsWithAttributeDash() {
Document doc = Jsoup.parse("<meta http-equiv=content-type value=utf8 id=1> <meta name=foo content=bar id=2> <div http-equiv=content-type value=utf8 id=3>");
Elements meta = doc.select("meta[http-equiv=content-type], meta[charset]");
assertEquals(1, meta.size());
assertEquals("1", meta.first().id());
}
@Test public void testGetElementsWithAttributeValue() {
Document doc = Jsoup.parse("<div style='bold'><p><p><b style></b></p></div>");
List<Element> els = doc.getElementsByAttributeValue("style", "bold");
assertEquals(1, els.size());
assertEquals("div", els.get(0).tagName());
List<Element> none = doc.getElementsByAttributeValue("style", "none");
assertEquals(0, none.size());
}
@Test public void testClassDomMethods() {
Document doc = Jsoup.parse("<div><span class=' mellow yellow '>Hello <b>Yellow</b></span></div>");
List<Element> els = doc.getElementsByAttribute("class");
Element span = els.get(0);
assertEquals("mellow yellow", span.className());
assertTrue(span.hasClass("mellow"));
assertTrue(span.hasClass("yellow"));
Set<String> classes = span.classNames();
assertEquals(2, classes.size());
assertTrue(classes.contains("mellow"));
assertTrue(classes.contains("yellow"));
assertEquals("", doc.className());
classes = doc.classNames();
assertEquals(0, classes.size());
assertFalse(doc.hasClass("mellow"));
}
@Test public void testClassUpdates() {
Document doc = Jsoup.parse("<div class='mellow yellow'></div>");
Element div = doc.select("div").first();
div.addClass("green");
assertEquals("mellow yellow green", div.className());
div.removeClass("red"); // noop
div.removeClass("yellow");
assertEquals("mellow green", div.className());
div.toggleClass("green").toggleClass("red");
assertEquals("mellow red", div.className());
}
@Test public void testOuterHtml() {
Document doc = Jsoup.parse("<div title='Tags &c.'><img src=foo.png><p><!-- comment -->Hello<p>there");
assertEquals("<html><head></head><body><div title=\"Tags &c.\"><img src=\"foo.png\"><p><!-- comment -->Hello</p><p>there</p></div></body></html>",
TextUtil.stripNewlines(doc.outerHtml()));
}
@Test public void testInnerHtml() {
Document doc = Jsoup.parse("<div>\n <p>Hello</p> </div>");
assertEquals("<p>Hello</p>", doc.getElementsByTag("div").get(0).html());
}
@Test public void testFormatHtml() {
Document doc = Jsoup.parse("<title>Format test</title><div><p>Hello <span>jsoup <span>users</span></span></p><p>Good.</p></div>");
assertEquals("<html>\n <head>\n <title>Format test</title>\n </head>\n <body>\n <div>\n <p>Hello <span>jsoup <span>users</span></span></p>\n <p>Good.</p>\n </div>\n </body>\n</html>", doc.html());
}
@Test public void testFormatOutline() {
Document doc = Jsoup.parse("<title>Format test</title><div><p>Hello <span>jsoup <span>users</span></span></p><p>Good.</p></div>");
doc.outputSettings().outline(true);
assertEquals("<html>\n <head>\n <title>Format test</title>\n </head>\n <body>\n <div>\n <p>\n Hello \n <span>\n jsoup \n <span>users</span>\n </span>\n </p>\n <p>Good.</p>\n </div>\n </body>\n</html>", doc.html());
}
@Test public void testSetIndent() {
Document doc = Jsoup.parse("<div><p>Hello\nthere</p></div>");
doc.outputSettings().indentAmount(0);
assertEquals("<html>\n<head></head>\n<body>\n<div>\n<p>Hello there</p>\n</div>\n</body>\n</html>", doc.html());
}
@Test public void testNotPretty() {
Document doc = Jsoup.parse("<div> \n<p>Hello\n there\n</p></div>");
doc.outputSettings().prettyPrint(false);
assertEquals("<html><head></head><body><div> \n<p>Hello\n there\n</p></div></body></html>", doc.html());
Element div = doc.select("div").first();
assertEquals(" \n<p>Hello\n there\n</p>", div.html());
}
@Test public void testEmptyElementFormatHtml() {
// don't put newlines into empty blocks
Document doc = Jsoup.parse("<section><div></div></section>");
assertEquals("<section>\n <div></div>\n</section>", doc.select("section").first().outerHtml());
}
@Test public void testNoIndentOnScriptAndStyle() {
// don't newline+indent closing </script> and </style> tags
Document doc = Jsoup.parse("<script>one\ntwo</script>\n<style>three\nfour</style>");
assertEquals("<script>one\ntwo</script> \n<style>three\nfour</style>", doc.head().html());
}
@Test public void testContainerOutput() {
Document doc = Jsoup.parse("<title>Hello there</title> <div><p>Hello</p><p>there</p></div> <div>Another</div>");
assertEquals("<title>Hello there</title>", doc.select("title").first().outerHtml());
assertEquals("<div>\n <p>Hello</p>\n <p>there</p>\n</div>", doc.select("div").first().outerHtml());
assertEquals("<div>\n <p>Hello</p>\n <p>there</p>\n</div> \n<div>\n Another\n</div>", doc.select("body").first().html());
}
@Test public void testSetText() {
String h = "<div id=1>Hello <p>there <b>now</b></p></div>";
Document doc = Jsoup.parse(h);
assertEquals("Hello there now", doc.text()); // need to sort out node whitespace
assertEquals("there now", doc.select("p").get(0).text());
Element div = doc.getElementById("1").text("Gone");
assertEquals("Gone", div.text());
assertEquals(0, doc.select("p").size());
}
@Test public void testAddNewElement() {
Document doc = Jsoup.parse("<div id=1><p>Hello</p></div>");
Element div = doc.getElementById("1");
div.appendElement("p").text("there");
div.appendElement("P").attr("class", "second").text("now");
assertEquals("<html><head></head><body><div id=\"1\"><p>Hello</p><p>there</p><p class=\"second\">now</p></div></body></html>",
TextUtil.stripNewlines(doc.html()));
// check sibling index (with short circuit on reindexChildren):
Elements ps = doc.select("p");
for (int i = 0; i < ps.size(); i++) {
assertEquals(i, ps.get(i).siblingIndex);
}
}
@Test public void testAppendRowToTable() {
Document doc = Jsoup.parse("<table><tr><td>1</td></tr></table>");
Element table = doc.select("tbody").first();
table.append("<tr><td>2</td></tr>");
assertEquals("<table><tbody><tr><td>1</td></tr><tr><td>2</td></tr></tbody></table>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void testPrependRowToTable() {
Document doc = Jsoup.parse("<table><tr><td>1</td></tr></table>");
Element table = doc.select("tbody").first();
table.prepend("<tr><td>2</td></tr>");
assertEquals("<table><tbody><tr><td>2</td></tr><tr><td>1</td></tr></tbody></table>", TextUtil.stripNewlines(doc.body().html()));
// check sibling index (reindexChildren):
Elements ps = doc.select("tr");
for (int i = 0; i < ps.size(); i++) {
assertEquals(i, ps.get(i).siblingIndex);
}
}
@Test public void testPrependElement() {
Document doc = Jsoup.parse("<div id=1><p>Hello</p></div>");
Element div = doc.getElementById("1");
div.prependElement("p").text("Before");
assertEquals("Before", div.child(0).text());
assertEquals("Hello", div.child(1).text());
}
@Test public void testAddNewText() {
Document doc = Jsoup.parse("<div id=1><p>Hello</p></div>");
Element div = doc.getElementById("1");
div.appendText(" there & now >");
assertEquals("<p>Hello</p> there & now >", TextUtil.stripNewlines(div.html()));
}
@Test public void testPrependText() {
Document doc = Jsoup.parse("<div id=1><p>Hello</p></div>");
Element div = doc.getElementById("1");
div.prependText("there & now > ");
assertEquals("there & now > Hello", div.text());
assertEquals("there & now > <p>Hello</p>", TextUtil.stripNewlines(div.html()));
}
@Test public void testAddNewHtml() {
Document doc = Jsoup.parse("<div id=1><p>Hello</p></div>");
Element div = doc.getElementById("1");
div.append("<p>there</p><p>now</p>");
assertEquals("<p>Hello</p><p>there</p><p>now</p>", TextUtil.stripNewlines(div.html()));
// check sibling index (no reindexChildren):
Elements ps = doc.select("p");
for (int i = 0; i < ps.size(); i++) {
assertEquals(i, ps.get(i).siblingIndex);
}
}
@Test public void testPrependNewHtml() {
Document doc = Jsoup.parse("<div id=1><p>Hello</p></div>");
Element div = doc.getElementById("1");
div.prepend("<p>there</p><p>now</p>");
assertEquals("<p>there</p><p>now</p><p>Hello</p>", TextUtil.stripNewlines(div.html()));
// check sibling index (reindexChildren):
Elements ps = doc.select("p");
for (int i = 0; i < ps.size(); i++) {
assertEquals(i, ps.get(i).siblingIndex);
}
}
@Test public void testSetHtml() {
Document doc = Jsoup.parse("<div id=1><p>Hello</p></div>");
Element div = doc.getElementById("1");
div.html("<p>there</p><p>now</p>");
assertEquals("<p>there</p><p>now</p>", TextUtil.stripNewlines(div.html()));
}
@Test public void testSetHtmlTitle() {
Document doc = Jsoup.parse("<html><head id=2><title id=1></title></head></html>");
Element title = doc.getElementById("1");
title.html("good");
assertEquals("good", title.html());
title.html("<i>bad</i>");
assertEquals("<i>bad</i>", title.html());
Element head = doc.getElementById("2");
head.html("<title><i>bad</i></title>");
assertEquals("<title><i>bad</i></title>", head.html());
}
@Test public void testWrap() {
Document doc = Jsoup.parse("<div><p>Hello</p><p>There</p></div>");
Element p = doc.select("p").first();
p.wrap("<div class='head'></div>");
assertEquals("<div><div class=\"head\"><p>Hello</p></div><p>There</p></div>", TextUtil.stripNewlines(doc.body().html()));
Element ret = p.wrap("<div><div class=foo></div><p>What?</p></div>");
assertEquals("<div><div class=\"head\"><div><div class=\"foo\"><p>Hello</p></div><p>What?</p></div></div><p>There</p></div>",
TextUtil.stripNewlines(doc.body().html()));
assertEquals(ret, p);
}
@Test public void before() {
Document doc = Jsoup.parse("<div><p>Hello</p><p>There</p></div>");
Element p1 = doc.select("p").first();
p1.before("<div>one</div><div>two</div>");
assertEquals("<div><div>one</div><div>two</div><p>Hello</p><p>There</p></div>", TextUtil.stripNewlines(doc.body().html()));
doc.select("p").last().before("<p>Three</p><!-- four -->");
assertEquals("<div><div>one</div><div>two</div><p>Hello</p><p>Three</p><!-- four --><p>There</p></div>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void after() {
Document doc = Jsoup.parse("<div><p>Hello</p><p>There</p></div>");
Element p1 = doc.select("p").first();
p1.after("<div>one</div><div>two</div>");
assertEquals("<div><p>Hello</p><div>one</div><div>two</div><p>There</p></div>", TextUtil.stripNewlines(doc.body().html()));
doc.select("p").last().after("<p>Three</p><!-- four -->");
assertEquals("<div><p>Hello</p><div>one</div><div>two</div><p>There</p><p>Three</p><!-- four --></div>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void testWrapWithRemainder() {
Document doc = Jsoup.parse("<div><p>Hello</p></div>");
Element p = doc.select("p").first();
p.wrap("<div class='head'></div><p>There!</p>");
assertEquals("<div><div class=\"head\"><p>Hello</p><p>There!</p></div></div>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void testHasText() {
Document doc = Jsoup.parse("<div><p>Hello</p><p></p></div>");
Element div = doc.select("div").first();
Elements ps = doc.select("p");
assertTrue(div.hasText());
assertTrue(ps.first().hasText());
assertFalse(ps.last().hasText());
}
@Test public void dataset() {
Document doc = Jsoup.parse("<div id=1 data-name=jsoup class=new data-package=jar>Hello</div><p id=2>Hello</p>");
Element div = doc.select("div").first();
Map<String, String> dataset = div.dataset();
Attributes attributes = div.attributes();
// size, get, set, add, remove
assertEquals(2, dataset.size());
assertEquals("jsoup", dataset.get("name"));
assertEquals("jar", dataset.get("package"));
dataset.put("name", "jsoup updated");
dataset.put("language", "java");
dataset.remove("package");
assertEquals(2, dataset.size());
assertEquals(4, attributes.size());
assertEquals("jsoup updated", attributes.get("data-name"));
assertEquals("jsoup updated", dataset.get("name"));
assertEquals("java", attributes.get("data-language"));
assertEquals("java", dataset.get("language"));
attributes.put("data-food", "bacon");
assertEquals(3, dataset.size());
assertEquals("bacon", dataset.get("food"));
attributes.put("data-", "empty");
assertEquals(null, dataset.get("")); // data- is not a data attribute
Element p = doc.select("p").first();
assertEquals(0, p.dataset().size());
}
@Test public void parentlessToString() {
Document doc = Jsoup.parse("<img src='foo'>");
Element img = doc.select("img").first();
assertEquals("<img src=\"foo\">", img.toString());
img.remove(); // lost its parent
assertEquals("<img src=\"foo\">", img.toString());
}
@Test public void testClone() {
Document doc = Jsoup.parse("<div><p>One<p><span>Two</div>");
Element p = doc.select("p").get(1);
Element clone = p.clone();
assertNull(clone.parent()); // should be orphaned
assertEquals(0, clone.siblingIndex);
assertEquals(1, p.siblingIndex);
assertNotNull(p.parent());
clone.append("<span>Three");
assertEquals("<p><span>Two</span><span>Three</span></p>", TextUtil.stripNewlines(clone.outerHtml()));
assertEquals("<div><p>One</p><p><span>Two</span></p></div>", TextUtil.stripNewlines(doc.body().html())); // not modified
doc.body().appendChild(clone); // adopt
assertNotNull(clone.parent());
assertEquals("<div><p>One</p><p><span>Two</span></p></div><p><span>Two</span><span>Three</span></p>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void testClonesClassnames() {
Document doc = Jsoup.parse("<div class='one two'></div>");
Element div = doc.select("div").first();
Set<String> classes = div.classNames();
assertEquals(2, classes.size());
assertTrue(classes.contains("one"));
assertTrue(classes.contains("two"));
Element copy = div.clone();
Set<String> copyClasses = copy.classNames();
assertEquals(2, copyClasses.size());
assertTrue(copyClasses.contains("one"));
assertTrue(copyClasses.contains("two"));
copyClasses.add("three");
copyClasses.remove("one");
assertTrue(classes.contains("one"));
assertFalse(classes.contains("three"));
assertFalse(copyClasses.contains("one"));
assertTrue(copyClasses.contains("three"));
assertEquals("", div.html());
assertEquals("", copy.html());
}
@Test public void testTagNameSet() {
Document doc = Jsoup.parse("<div><i>Hello</i>");
doc.select("i").first().tagName("em");
assertEquals(0, doc.select("i").size());
assertEquals(1, doc.select("em").size());
assertEquals("<em>Hello</em>", doc.select("div").first().html());
}
@Test public void testHtmlContainsOuter() {
Document doc = Jsoup.parse("<title>Check</title> <div>Hello there</div>");
doc.outputSettings().indentAmount(0);
assertTrue(doc.html().contains(doc.select("title").outerHtml()));
assertTrue(doc.html().contains(doc.select("div").outerHtml()));
}
@Test public void testGetTextNodes() {
Document doc = Jsoup.parse("<p>One <span>Two</span> Three <br> Four</p>");
List<TextNode> textNodes = doc.select("p").first().textNodes();
assertEquals(3, textNodes.size());
assertEquals("One ", textNodes.get(0).text());
assertEquals(" Three ", textNodes.get(1).text());
assertEquals(" Four", textNodes.get(2).text());
assertEquals(0, doc.select("br").first().textNodes().size());
}
@Test public void testManipulateTextNodes() {
Document doc = Jsoup.parse("<p>One <span>Two</span> Three <br> Four</p>");
Element p = doc.select("p").first();
List<TextNode> textNodes = p.textNodes();
textNodes.get(1).text(" three-more ");
textNodes.get(2).splitText(3).text("-ur");
assertEquals("One Two three-more Fo-ur", p.text());
assertEquals("One three-more Fo-ur", p.ownText());
assertEquals(4, p.textNodes().size()); // grew because of split
}
@Test public void testGetDataNodes() {
Document doc = Jsoup.parse("<script>One Two</script> <style>Three Four</style> <p>Fix Six</p>");
Element script = doc.select("script").first();
Element style = doc.select("style").first();
Element p = doc.select("p").first();
List<DataNode> scriptData = script.dataNodes();
assertEquals(1, scriptData.size());
assertEquals("One Two", scriptData.get(0).getWholeData());
List<DataNode> styleData = style.dataNodes();
assertEquals(1, styleData.size());
assertEquals("Three Four", styleData.get(0).getWholeData());
List<DataNode> pData = p.dataNodes();
assertEquals(0, pData.size());
}
@Test public void elementIsNotASiblingOfItself() {
Document doc = Jsoup.parse("<div><p>One<p>Two<p>Three</div>");
Element p2 = doc.select("p").get(1);
assertEquals("Two", p2.text());
Elements els = p2.siblingElements();
assertEquals(2, els.size());
assertEquals("<p>One</p>", els.get(0).outerHtml());
assertEquals("<p>Three</p>", els.get(1).outerHtml());
}
@Test public void testChildThrowsIndexOutOfBoundsOnMissing() {
Document doc = Jsoup.parse("<div><p>One</p><p>Two</p></div>");
Element div = doc.select("div").first();
assertEquals(2, div.children().size());
assertEquals("One", div.child(0).text());
try {
div.child(3);
fail("Should throw index out of bounds");
} catch (IndexOutOfBoundsException e) {}
}
@Test
public void moveByAppend() {
// test for https://github.com/jhy/jsoup/issues/239
// can empty an element and append its children to another element
Document doc = Jsoup.parse("<div id=1>Text <p>One</p> Text <p>Two</p></div><div id=2></div>");
Element div1 = doc.select("div").get(0);
Element div2 = doc.select("div").get(1);
assertEquals(4, div1.childNodeSize());
List<Node> children = div1.childNodes();
assertEquals(4, children.size());
div2.insertChildren(0, children);
assertEquals(0, children.size()); // children is backed by div1.childNodes, moved, so should be 0 now
assertEquals(0, div1.childNodeSize());
assertEquals(4, div2.childNodeSize());
assertEquals("<div id=\"1\"></div>\n<div id=\"2\">\n Text \n <p>One</p> Text \n <p>Two</p>\n</div>",
doc.body().html());
}
@Test
public void insertChildrenArgumentValidation() {
Document doc = Jsoup.parse("<div id=1>Text <p>One</p> Text <p>Two</p></div><div id=2></div>");
Element div1 = doc.select("div").get(0);
Element div2 = doc.select("div").get(1);
List<Node> children = div1.childNodes();
try {
div2.insertChildren(6, children);
fail();
} catch (IllegalArgumentException e) {}
try {
div2.insertChildren(-5, children);
fail();
} catch (IllegalArgumentException e) {
}
try {
div2.insertChildren(0, null);
fail();
} catch (IllegalArgumentException e) {
}
}
@Test
public void insertChildrenAtPosition() {
Document doc = Jsoup.parse("<div id=1>Text1 <p>One</p> Text2 <p>Two</p></div><div id=2>Text3 <p>Three</p></div>");
Element div1 = doc.select("div").get(0);
Elements p1s = div1.select("p");
Element div2 = doc.select("div").get(1);
assertEquals(2, div2.childNodeSize());
div2.insertChildren(-1, p1s);
assertEquals(2, div1.childNodeSize()); // moved two out
assertEquals(4, div2.childNodeSize());
assertEquals(3, p1s.get(1).siblingIndex()); // should be last
List<Node> els = new ArrayList<Node>();
Element el1 = new Element(Tag.valueOf("span"), "").text("Span1");
Element el2 = new Element(Tag.valueOf("span"), "").text("Span2");
TextNode tn1 = new TextNode("Text4", "");
els.add(el1);
els.add(el2);
els.add(tn1);
assertNull(el1.parent());
div2.insertChildren(-2, els);
assertEquals(div2, el1.parent());
assertEquals(7, div2.childNodeSize());
assertEquals(3, el1.siblingIndex());
assertEquals(4, el2.siblingIndex());
assertEquals(5, tn1.siblingIndex());
}
@Test
public void insertChildrenAsCopy() {
Document doc = Jsoup.parse("<div id=1>Text <p>One</p> Text <p>Two</p></div><div id=2></div>");
Element div1 = doc.select("div").get(0);
Element div2 = doc.select("div").get(1);
Elements ps = doc.select("p").clone();
ps.first().text("One cloned");
div2.insertChildren(-1, ps);
assertEquals(4, div1.childNodeSize()); // not moved -- cloned
assertEquals(2, div2.childNodeSize());
assertEquals("<div id=\"1\">Text <p>One</p> Text <p>Two</p></div><div id=\"2\"><p>One cloned</p><p>Two</p></div>",
TextUtil.stripNewlines(doc.body().html()));
}
@Test
public void testCssPath() {
Document doc = Jsoup.parse("<div id=\"id1\">A</div><div>B</div><div class=\"c1 c2\">C</div>");
Element divA = doc.select("div").get(0);
Element divB = doc.select("div").get(1);
Element divC = doc.select("div").get(2);
assertEquals(divA.cssSelector(), "#id1");
assertEquals(divB.cssSelector(), "html > body > div:nth-child(2)");
assertEquals(divC.cssSelector(), "html > body > div.c1.c2");
assertTrue(divA == doc.select(divA.cssSelector()).first());
assertTrue(divB == doc.select(divB.cssSelector()).first());
assertTrue(divC == doc.select(divC.cssSelector()).first());
}
@Test
public void testClassNames() {
Document doc = Jsoup.parse("<div class=\"c1 c2\">C</div>");
Element div = doc.select("div").get(0);
assertEquals("c1 c2", div.className());
final Set<String> set1 = div.classNames();
final Object[] arr1 = set1.toArray();
assertTrue(arr1.length==2);
assertEquals("c1", arr1[0]);
assertEquals("c2", arr1[1]);
// Changes to the set should not be reflected in the Elements getters
set1.add("c3");
assertTrue(2==div.classNames().size());
assertEquals("c1 c2", div.className());
// Update the class names to a fresh set
final Set<String> newSet = new LinkedHashSet<String>(3);
newSet.addAll(set1);
newSet.add("c3");
div.classNames(newSet);
assertEquals("c1 c2 c3", div.className());
final Set<String> set2 = div.classNames();
final Object[] arr2 = set2.toArray();
assertTrue(arr2.length==3);
assertEquals("c1", arr2[0]);
assertEquals("c2", arr2[1]);
assertEquals("c3", arr2[2]);
}
@Test
public void testHashAndEquals() {
String doc1 = "<div id=1><p class=one>One</p><p class=one>One</p><p class=one>Two</p><p class=two>One</p></div>" +
"<div id=2><p class=one>One</p><p class=one>One</p><p class=one>Two</p><p class=two>One</p></div>";
Document doc = Jsoup.parse(doc1);
Elements els = doc.select("p");
/*
for (Element el : els) {
System.out.println(el.hashCode() + " - " + el.outerHtml());
}
0 1534787905 - <p class="one">One</p>
1 1534787905 - <p class="one">One</p>
2 1539683239 - <p class="one">Two</p>
3 1535455211 - <p class="two">One</p>
4 1534787905 - <p class="one">One</p>
5 1534787905 - <p class="one">One</p>
6 1539683239 - <p class="one">Two</p>
7 1535455211 - <p class="two">One</p>
*/
assertEquals(8, els.size());
Element e0 = els.get(0);
Element e1 = els.get(1);
Element e2 = els.get(2);
Element e3 = els.get(3);
Element e4 = els.get(4);
Element e5 = els.get(5);
Element e6 = els.get(6);
Element e7 = els.get(7);
assertEquals(e0, e1);
assertEquals(e0, e4);
assertEquals(e0, e5);
assertFalse(e0.equals(e2));
assertFalse(e0.equals(e3));
assertFalse(e0.equals(e6));
assertFalse(e0.equals(e7));
assertEquals(e0.hashCode(), e1.hashCode());
assertEquals(e0.hashCode(), e4.hashCode());
assertEquals(e0.hashCode(), e5.hashCode());
assertFalse(e0.hashCode() == (e2.hashCode()));
assertFalse(e0.hashCode() == (e3).hashCode());
assertFalse(e0.hashCode() == (e6).hashCode());
assertFalse(e0.hashCode() == (e7).hashCode());
}
} | // You are a professional Java test case writer, please create a test case named `testHashAndEquals` for the issue `Jsoup-537`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Jsoup-537
//
// ## Issue-Title:
// Element.hashCode() ignores the content text of the element.
//
// ## Issue-Description:
// Found [this question](http://stackoverflow.com/questions/28970732/jsoup-node-hash-code-collision-when-traversing-dom-tree/28971463) on SO, OP was using `Element.hashCode()` and it wasn't woring right.
//
//
// The problem is that when jsoup generates the hashCode of an Element, the content text of the element will be ignored, and the hashCode is generated only based on the attributes, and the hashCode of the parent Element.
//
//
//
//
// ---
//
//
// Using the following HTML:
//
//
//
// ```
// <html>
// <head>
// </head>
// <body>
// <div style="blah">TODO: write content</div>
// <div style="blah">Nothing here</div>
// <p style="test">Empty</p>
// <p style="nothing">Empty</p>
// </body>
// </html>
//
// ```
//
// And the following code:
//
//
//
// ```
// String html = //HTML posted above
//
// Document doc = Jsoup.parse(html);
//
// Elements elements = doc.select("[style]");
// for (Element e : elements) {
// System.out.println(e.hashCode());
// }
//
// ```
//
// It gives:
//
//
//
// ```
// -148184373
// -148184373
// -1050420242
// 2013043377
//
// ```
//
// I believe the hashCode should be different for the first two Elements, since the content is text is different. Or is this intended behaviour?
//
//
//
//
@Test
public void testHashAndEquals() {
| 799 | 41 | 752 | src/test/java/org/jsoup/nodes/ElementTest.java | src/test/java | ```markdown
## Issue-ID: Jsoup-537
## Issue-Title:
Element.hashCode() ignores the content text of the element.
## Issue-Description:
Found [this question](http://stackoverflow.com/questions/28970732/jsoup-node-hash-code-collision-when-traversing-dom-tree/28971463) on SO, OP was using `Element.hashCode()` and it wasn't woring right.
The problem is that when jsoup generates the hashCode of an Element, the content text of the element will be ignored, and the hashCode is generated only based on the attributes, and the hashCode of the parent Element.
---
Using the following HTML:
```
<html>
<head>
</head>
<body>
<div style="blah">TODO: write content</div>
<div style="blah">Nothing here</div>
<p style="test">Empty</p>
<p style="nothing">Empty</p>
</body>
</html>
```
And the following code:
```
String html = //HTML posted above
Document doc = Jsoup.parse(html);
Elements elements = doc.select("[style]");
for (Element e : elements) {
System.out.println(e.hashCode());
}
```
It gives:
```
-148184373
-148184373
-1050420242
2013043377
```
I believe the hashCode should be different for the first two Elements, since the content is text is different. Or is this intended behaviour?
```
You are a professional Java test case writer, please create a test case named `testHashAndEquals` for the issue `Jsoup-537`, utilizing the provided issue report information and the following function signature.
```java
@Test
public void testHashAndEquals() {
```
| 752 | [
"org.jsoup.nodes.Element"
] | 9e8c440979d956df0123231d6dcab3996a63b8c8c7260b6d3d90b5ff6093f5e3 | @Test
public void testHashAndEquals() | // You are a professional Java test case writer, please create a test case named `testHashAndEquals` for the issue `Jsoup-537`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Jsoup-537
//
// ## Issue-Title:
// Element.hashCode() ignores the content text of the element.
//
// ## Issue-Description:
// Found [this question](http://stackoverflow.com/questions/28970732/jsoup-node-hash-code-collision-when-traversing-dom-tree/28971463) on SO, OP was using `Element.hashCode()` and it wasn't woring right.
//
//
// The problem is that when jsoup generates the hashCode of an Element, the content text of the element will be ignored, and the hashCode is generated only based on the attributes, and the hashCode of the parent Element.
//
//
//
//
// ---
//
//
// Using the following HTML:
//
//
//
// ```
// <html>
// <head>
// </head>
// <body>
// <div style="blah">TODO: write content</div>
// <div style="blah">Nothing here</div>
// <p style="test">Empty</p>
// <p style="nothing">Empty</p>
// </body>
// </html>
//
// ```
//
// And the following code:
//
//
//
// ```
// String html = //HTML posted above
//
// Document doc = Jsoup.parse(html);
//
// Elements elements = doc.select("[style]");
// for (Element e : elements) {
// System.out.println(e.hashCode());
// }
//
// ```
//
// It gives:
//
//
//
// ```
// -148184373
// -148184373
// -1050420242
// 2013043377
//
// ```
//
// I believe the hashCode should be different for the first two Elements, since the content is text is different. Or is this intended behaviour?
//
//
//
//
| Jsoup | package org.jsoup.nodes;
import org.jsoup.Jsoup;
import org.jsoup.TextUtil;
import org.jsoup.helper.StringUtil;
import org.jsoup.parser.Tag;
import org.jsoup.select.Elements;
import org.junit.Test;
import static org.junit.Assert.*;
import java.util.ArrayList;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Set;
import java.util.Map;
/**
* Tests for Element (DOM stuff mostly).
*
* @author Jonathan Hedley
*/
public class ElementTest {
private String reference = "<div id=div1><p>Hello</p><p>Another <b>element</b></p><div id=div2><img src=foo.png></div></div>";
@Test public void getElementsByTagName() {
Document doc = Jsoup.parse(reference);
List<Element> divs = doc.getElementsByTag("div");
assertEquals(2, divs.size());
assertEquals("div1", divs.get(0).id());
assertEquals("div2", divs.get(1).id());
List<Element> ps = doc.getElementsByTag("p");
assertEquals(2, ps.size());
assertEquals("Hello", ((TextNode) ps.get(0).childNode(0)).getWholeText());
assertEquals("Another ", ((TextNode) ps.get(1).childNode(0)).getWholeText());
List<Element> ps2 = doc.getElementsByTag("P");
assertEquals(ps, ps2);
List<Element> imgs = doc.getElementsByTag("img");
assertEquals("foo.png", imgs.get(0).attr("src"));
List<Element> empty = doc.getElementsByTag("wtf");
assertEquals(0, empty.size());
}
@Test public void getNamespacedElementsByTag() {
Document doc = Jsoup.parse("<div><abc:def id=1>Hello</abc:def></div>");
Elements els = doc.getElementsByTag("abc:def");
assertEquals(1, els.size());
assertEquals("1", els.first().id());
assertEquals("abc:def", els.first().tagName());
}
@Test public void testGetElementById() {
Document doc = Jsoup.parse(reference);
Element div = doc.getElementById("div1");
assertEquals("div1", div.id());
assertNull(doc.getElementById("none"));
Document doc2 = Jsoup.parse("<div id=1><div id=2><p>Hello <span id=2>world!</span></p></div></div>");
Element div2 = doc2.getElementById("2");
assertEquals("div", div2.tagName()); // not the span
Element span = div2.child(0).getElementById("2"); // called from <p> context should be span
assertEquals("span", span.tagName());
}
@Test public void testGetText() {
Document doc = Jsoup.parse(reference);
assertEquals("Hello Another element", doc.text());
assertEquals("Another element", doc.getElementsByTag("p").get(1).text());
}
@Test public void testGetChildText() {
Document doc = Jsoup.parse("<p>Hello <b>there</b> now");
Element p = doc.select("p").first();
assertEquals("Hello there now", p.text());
assertEquals("Hello now", p.ownText());
}
@Test public void testNormalisesText() {
String h = "<p>Hello<p>There.</p> \n <p>Here <b>is</b> \n s<b>om</b>e text.";
Document doc = Jsoup.parse(h);
String text = doc.text();
assertEquals("Hello There. Here is some text.", text);
}
@Test public void testKeepsPreText() {
String h = "<p>Hello \n \n there.</p> <div><pre> What's \n\n that?</pre>";
Document doc = Jsoup.parse(h);
assertEquals("Hello there. What's \n\n that?", doc.text());
}
@Test public void testKeepsPreTextInCode() {
String h = "<pre><code>code\n\ncode</code></pre>";
Document doc = Jsoup.parse(h);
assertEquals("code\n\ncode", doc.text());
assertEquals("<pre><code>code\n\ncode</code></pre>", doc.body().html());
}
@Test public void testBrHasSpace() {
Document doc = Jsoup.parse("<p>Hello<br>there</p>");
assertEquals("Hello there", doc.text());
assertEquals("Hello there", doc.select("p").first().ownText());
doc = Jsoup.parse("<p>Hello <br> there</p>");
assertEquals("Hello there", doc.text());
}
@Test public void testGetSiblings() {
Document doc = Jsoup.parse("<div><p>Hello<p id=1>there<p>this<p>is<p>an<p id=last>element</div>");
Element p = doc.getElementById("1");
assertEquals("there", p.text());
assertEquals("Hello", p.previousElementSibling().text());
assertEquals("this", p.nextElementSibling().text());
assertEquals("Hello", p.firstElementSibling().text());
assertEquals("element", p.lastElementSibling().text());
}
@Test public void testGetParents() {
Document doc = Jsoup.parse("<div><p>Hello <span>there</span></div>");
Element span = doc.select("span").first();
Elements parents = span.parents();
assertEquals(4, parents.size());
assertEquals("p", parents.get(0).tagName());
assertEquals("div", parents.get(1).tagName());
assertEquals("body", parents.get(2).tagName());
assertEquals("html", parents.get(3).tagName());
}
@Test public void testElementSiblingIndex() {
Document doc = Jsoup.parse("<div><p>One</p>...<p>Two</p>...<p>Three</p>");
Elements ps = doc.select("p");
assertTrue(0 == ps.get(0).elementSiblingIndex());
assertTrue(1 == ps.get(1).elementSiblingIndex());
assertTrue(2 == ps.get(2).elementSiblingIndex());
}
@Test public void testGetElementsWithClass() {
Document doc = Jsoup.parse("<div class='mellow yellow'><span class=mellow>Hello <b class='yellow'>Yellow!</b></span><p>Empty</p></div>");
List<Element> els = doc.getElementsByClass("mellow");
assertEquals(2, els.size());
assertEquals("div", els.get(0).tagName());
assertEquals("span", els.get(1).tagName());
List<Element> els2 = doc.getElementsByClass("yellow");
assertEquals(2, els2.size());
assertEquals("div", els2.get(0).tagName());
assertEquals("b", els2.get(1).tagName());
List<Element> none = doc.getElementsByClass("solo");
assertEquals(0, none.size());
}
@Test public void testGetElementsWithAttribute() {
Document doc = Jsoup.parse("<div style='bold'><p title=qux><p><b style></b></p></div>");
List<Element> els = doc.getElementsByAttribute("style");
assertEquals(2, els.size());
assertEquals("div", els.get(0).tagName());
assertEquals("b", els.get(1).tagName());
List<Element> none = doc.getElementsByAttribute("class");
assertEquals(0, none.size());
}
@Test public void testGetElementsWithAttributeDash() {
Document doc = Jsoup.parse("<meta http-equiv=content-type value=utf8 id=1> <meta name=foo content=bar id=2> <div http-equiv=content-type value=utf8 id=3>");
Elements meta = doc.select("meta[http-equiv=content-type], meta[charset]");
assertEquals(1, meta.size());
assertEquals("1", meta.first().id());
}
@Test public void testGetElementsWithAttributeValue() {
Document doc = Jsoup.parse("<div style='bold'><p><p><b style></b></p></div>");
List<Element> els = doc.getElementsByAttributeValue("style", "bold");
assertEquals(1, els.size());
assertEquals("div", els.get(0).tagName());
List<Element> none = doc.getElementsByAttributeValue("style", "none");
assertEquals(0, none.size());
}
@Test public void testClassDomMethods() {
Document doc = Jsoup.parse("<div><span class=' mellow yellow '>Hello <b>Yellow</b></span></div>");
List<Element> els = doc.getElementsByAttribute("class");
Element span = els.get(0);
assertEquals("mellow yellow", span.className());
assertTrue(span.hasClass("mellow"));
assertTrue(span.hasClass("yellow"));
Set<String> classes = span.classNames();
assertEquals(2, classes.size());
assertTrue(classes.contains("mellow"));
assertTrue(classes.contains("yellow"));
assertEquals("", doc.className());
classes = doc.classNames();
assertEquals(0, classes.size());
assertFalse(doc.hasClass("mellow"));
}
@Test public void testClassUpdates() {
Document doc = Jsoup.parse("<div class='mellow yellow'></div>");
Element div = doc.select("div").first();
div.addClass("green");
assertEquals("mellow yellow green", div.className());
div.removeClass("red"); // noop
div.removeClass("yellow");
assertEquals("mellow green", div.className());
div.toggleClass("green").toggleClass("red");
assertEquals("mellow red", div.className());
}
@Test public void testOuterHtml() {
Document doc = Jsoup.parse("<div title='Tags &c.'><img src=foo.png><p><!-- comment -->Hello<p>there");
assertEquals("<html><head></head><body><div title=\"Tags &c.\"><img src=\"foo.png\"><p><!-- comment -->Hello</p><p>there</p></div></body></html>",
TextUtil.stripNewlines(doc.outerHtml()));
}
@Test public void testInnerHtml() {
Document doc = Jsoup.parse("<div>\n <p>Hello</p> </div>");
assertEquals("<p>Hello</p>", doc.getElementsByTag("div").get(0).html());
}
@Test public void testFormatHtml() {
Document doc = Jsoup.parse("<title>Format test</title><div><p>Hello <span>jsoup <span>users</span></span></p><p>Good.</p></div>");
assertEquals("<html>\n <head>\n <title>Format test</title>\n </head>\n <body>\n <div>\n <p>Hello <span>jsoup <span>users</span></span></p>\n <p>Good.</p>\n </div>\n </body>\n</html>", doc.html());
}
@Test public void testFormatOutline() {
Document doc = Jsoup.parse("<title>Format test</title><div><p>Hello <span>jsoup <span>users</span></span></p><p>Good.</p></div>");
doc.outputSettings().outline(true);
assertEquals("<html>\n <head>\n <title>Format test</title>\n </head>\n <body>\n <div>\n <p>\n Hello \n <span>\n jsoup \n <span>users</span>\n </span>\n </p>\n <p>Good.</p>\n </div>\n </body>\n</html>", doc.html());
}
@Test public void testSetIndent() {
Document doc = Jsoup.parse("<div><p>Hello\nthere</p></div>");
doc.outputSettings().indentAmount(0);
assertEquals("<html>\n<head></head>\n<body>\n<div>\n<p>Hello there</p>\n</div>\n</body>\n</html>", doc.html());
}
@Test public void testNotPretty() {
Document doc = Jsoup.parse("<div> \n<p>Hello\n there\n</p></div>");
doc.outputSettings().prettyPrint(false);
assertEquals("<html><head></head><body><div> \n<p>Hello\n there\n</p></div></body></html>", doc.html());
Element div = doc.select("div").first();
assertEquals(" \n<p>Hello\n there\n</p>", div.html());
}
@Test public void testEmptyElementFormatHtml() {
// don't put newlines into empty blocks
Document doc = Jsoup.parse("<section><div></div></section>");
assertEquals("<section>\n <div></div>\n</section>", doc.select("section").first().outerHtml());
}
@Test public void testNoIndentOnScriptAndStyle() {
// don't newline+indent closing </script> and </style> tags
Document doc = Jsoup.parse("<script>one\ntwo</script>\n<style>three\nfour</style>");
assertEquals("<script>one\ntwo</script> \n<style>three\nfour</style>", doc.head().html());
}
@Test public void testContainerOutput() {
Document doc = Jsoup.parse("<title>Hello there</title> <div><p>Hello</p><p>there</p></div> <div>Another</div>");
assertEquals("<title>Hello there</title>", doc.select("title").first().outerHtml());
assertEquals("<div>\n <p>Hello</p>\n <p>there</p>\n</div>", doc.select("div").first().outerHtml());
assertEquals("<div>\n <p>Hello</p>\n <p>there</p>\n</div> \n<div>\n Another\n</div>", doc.select("body").first().html());
}
@Test public void testSetText() {
String h = "<div id=1>Hello <p>there <b>now</b></p></div>";
Document doc = Jsoup.parse(h);
assertEquals("Hello there now", doc.text()); // need to sort out node whitespace
assertEquals("there now", doc.select("p").get(0).text());
Element div = doc.getElementById("1").text("Gone");
assertEquals("Gone", div.text());
assertEquals(0, doc.select("p").size());
}
@Test public void testAddNewElement() {
Document doc = Jsoup.parse("<div id=1><p>Hello</p></div>");
Element div = doc.getElementById("1");
div.appendElement("p").text("there");
div.appendElement("P").attr("class", "second").text("now");
assertEquals("<html><head></head><body><div id=\"1\"><p>Hello</p><p>there</p><p class=\"second\">now</p></div></body></html>",
TextUtil.stripNewlines(doc.html()));
// check sibling index (with short circuit on reindexChildren):
Elements ps = doc.select("p");
for (int i = 0; i < ps.size(); i++) {
assertEquals(i, ps.get(i).siblingIndex);
}
}
@Test public void testAppendRowToTable() {
Document doc = Jsoup.parse("<table><tr><td>1</td></tr></table>");
Element table = doc.select("tbody").first();
table.append("<tr><td>2</td></tr>");
assertEquals("<table><tbody><tr><td>1</td></tr><tr><td>2</td></tr></tbody></table>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void testPrependRowToTable() {
Document doc = Jsoup.parse("<table><tr><td>1</td></tr></table>");
Element table = doc.select("tbody").first();
table.prepend("<tr><td>2</td></tr>");
assertEquals("<table><tbody><tr><td>2</td></tr><tr><td>1</td></tr></tbody></table>", TextUtil.stripNewlines(doc.body().html()));
// check sibling index (reindexChildren):
Elements ps = doc.select("tr");
for (int i = 0; i < ps.size(); i++) {
assertEquals(i, ps.get(i).siblingIndex);
}
}
@Test public void testPrependElement() {
Document doc = Jsoup.parse("<div id=1><p>Hello</p></div>");
Element div = doc.getElementById("1");
div.prependElement("p").text("Before");
assertEquals("Before", div.child(0).text());
assertEquals("Hello", div.child(1).text());
}
@Test public void testAddNewText() {
Document doc = Jsoup.parse("<div id=1><p>Hello</p></div>");
Element div = doc.getElementById("1");
div.appendText(" there & now >");
assertEquals("<p>Hello</p> there & now >", TextUtil.stripNewlines(div.html()));
}
@Test public void testPrependText() {
Document doc = Jsoup.parse("<div id=1><p>Hello</p></div>");
Element div = doc.getElementById("1");
div.prependText("there & now > ");
assertEquals("there & now > Hello", div.text());
assertEquals("there & now > <p>Hello</p>", TextUtil.stripNewlines(div.html()));
}
@Test public void testAddNewHtml() {
Document doc = Jsoup.parse("<div id=1><p>Hello</p></div>");
Element div = doc.getElementById("1");
div.append("<p>there</p><p>now</p>");
assertEquals("<p>Hello</p><p>there</p><p>now</p>", TextUtil.stripNewlines(div.html()));
// check sibling index (no reindexChildren):
Elements ps = doc.select("p");
for (int i = 0; i < ps.size(); i++) {
assertEquals(i, ps.get(i).siblingIndex);
}
}
@Test public void testPrependNewHtml() {
Document doc = Jsoup.parse("<div id=1><p>Hello</p></div>");
Element div = doc.getElementById("1");
div.prepend("<p>there</p><p>now</p>");
assertEquals("<p>there</p><p>now</p><p>Hello</p>", TextUtil.stripNewlines(div.html()));
// check sibling index (reindexChildren):
Elements ps = doc.select("p");
for (int i = 0; i < ps.size(); i++) {
assertEquals(i, ps.get(i).siblingIndex);
}
}
@Test public void testSetHtml() {
Document doc = Jsoup.parse("<div id=1><p>Hello</p></div>");
Element div = doc.getElementById("1");
div.html("<p>there</p><p>now</p>");
assertEquals("<p>there</p><p>now</p>", TextUtil.stripNewlines(div.html()));
}
@Test public void testSetHtmlTitle() {
Document doc = Jsoup.parse("<html><head id=2><title id=1></title></head></html>");
Element title = doc.getElementById("1");
title.html("good");
assertEquals("good", title.html());
title.html("<i>bad</i>");
assertEquals("<i>bad</i>", title.html());
Element head = doc.getElementById("2");
head.html("<title><i>bad</i></title>");
assertEquals("<title><i>bad</i></title>", head.html());
}
@Test public void testWrap() {
Document doc = Jsoup.parse("<div><p>Hello</p><p>There</p></div>");
Element p = doc.select("p").first();
p.wrap("<div class='head'></div>");
assertEquals("<div><div class=\"head\"><p>Hello</p></div><p>There</p></div>", TextUtil.stripNewlines(doc.body().html()));
Element ret = p.wrap("<div><div class=foo></div><p>What?</p></div>");
assertEquals("<div><div class=\"head\"><div><div class=\"foo\"><p>Hello</p></div><p>What?</p></div></div><p>There</p></div>",
TextUtil.stripNewlines(doc.body().html()));
assertEquals(ret, p);
}
@Test public void before() {
Document doc = Jsoup.parse("<div><p>Hello</p><p>There</p></div>");
Element p1 = doc.select("p").first();
p1.before("<div>one</div><div>two</div>");
assertEquals("<div><div>one</div><div>two</div><p>Hello</p><p>There</p></div>", TextUtil.stripNewlines(doc.body().html()));
doc.select("p").last().before("<p>Three</p><!-- four -->");
assertEquals("<div><div>one</div><div>two</div><p>Hello</p><p>Three</p><!-- four --><p>There</p></div>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void after() {
Document doc = Jsoup.parse("<div><p>Hello</p><p>There</p></div>");
Element p1 = doc.select("p").first();
p1.after("<div>one</div><div>two</div>");
assertEquals("<div><p>Hello</p><div>one</div><div>two</div><p>There</p></div>", TextUtil.stripNewlines(doc.body().html()));
doc.select("p").last().after("<p>Three</p><!-- four -->");
assertEquals("<div><p>Hello</p><div>one</div><div>two</div><p>There</p><p>Three</p><!-- four --></div>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void testWrapWithRemainder() {
Document doc = Jsoup.parse("<div><p>Hello</p></div>");
Element p = doc.select("p").first();
p.wrap("<div class='head'></div><p>There!</p>");
assertEquals("<div><div class=\"head\"><p>Hello</p><p>There!</p></div></div>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void testHasText() {
Document doc = Jsoup.parse("<div><p>Hello</p><p></p></div>");
Element div = doc.select("div").first();
Elements ps = doc.select("p");
assertTrue(div.hasText());
assertTrue(ps.first().hasText());
assertFalse(ps.last().hasText());
}
@Test public void dataset() {
Document doc = Jsoup.parse("<div id=1 data-name=jsoup class=new data-package=jar>Hello</div><p id=2>Hello</p>");
Element div = doc.select("div").first();
Map<String, String> dataset = div.dataset();
Attributes attributes = div.attributes();
// size, get, set, add, remove
assertEquals(2, dataset.size());
assertEquals("jsoup", dataset.get("name"));
assertEquals("jar", dataset.get("package"));
dataset.put("name", "jsoup updated");
dataset.put("language", "java");
dataset.remove("package");
assertEquals(2, dataset.size());
assertEquals(4, attributes.size());
assertEquals("jsoup updated", attributes.get("data-name"));
assertEquals("jsoup updated", dataset.get("name"));
assertEquals("java", attributes.get("data-language"));
assertEquals("java", dataset.get("language"));
attributes.put("data-food", "bacon");
assertEquals(3, dataset.size());
assertEquals("bacon", dataset.get("food"));
attributes.put("data-", "empty");
assertEquals(null, dataset.get("")); // data- is not a data attribute
Element p = doc.select("p").first();
assertEquals(0, p.dataset().size());
}
@Test public void parentlessToString() {
Document doc = Jsoup.parse("<img src='foo'>");
Element img = doc.select("img").first();
assertEquals("<img src=\"foo\">", img.toString());
img.remove(); // lost its parent
assertEquals("<img src=\"foo\">", img.toString());
}
@Test public void testClone() {
Document doc = Jsoup.parse("<div><p>One<p><span>Two</div>");
Element p = doc.select("p").get(1);
Element clone = p.clone();
assertNull(clone.parent()); // should be orphaned
assertEquals(0, clone.siblingIndex);
assertEquals(1, p.siblingIndex);
assertNotNull(p.parent());
clone.append("<span>Three");
assertEquals("<p><span>Two</span><span>Three</span></p>", TextUtil.stripNewlines(clone.outerHtml()));
assertEquals("<div><p>One</p><p><span>Two</span></p></div>", TextUtil.stripNewlines(doc.body().html())); // not modified
doc.body().appendChild(clone); // adopt
assertNotNull(clone.parent());
assertEquals("<div><p>One</p><p><span>Two</span></p></div><p><span>Two</span><span>Three</span></p>", TextUtil.stripNewlines(doc.body().html()));
}
@Test public void testClonesClassnames() {
Document doc = Jsoup.parse("<div class='one two'></div>");
Element div = doc.select("div").first();
Set<String> classes = div.classNames();
assertEquals(2, classes.size());
assertTrue(classes.contains("one"));
assertTrue(classes.contains("two"));
Element copy = div.clone();
Set<String> copyClasses = copy.classNames();
assertEquals(2, copyClasses.size());
assertTrue(copyClasses.contains("one"));
assertTrue(copyClasses.contains("two"));
copyClasses.add("three");
copyClasses.remove("one");
assertTrue(classes.contains("one"));
assertFalse(classes.contains("three"));
assertFalse(copyClasses.contains("one"));
assertTrue(copyClasses.contains("three"));
assertEquals("", div.html());
assertEquals("", copy.html());
}
@Test public void testTagNameSet() {
Document doc = Jsoup.parse("<div><i>Hello</i>");
doc.select("i").first().tagName("em");
assertEquals(0, doc.select("i").size());
assertEquals(1, doc.select("em").size());
assertEquals("<em>Hello</em>", doc.select("div").first().html());
}
@Test public void testHtmlContainsOuter() {
Document doc = Jsoup.parse("<title>Check</title> <div>Hello there</div>");
doc.outputSettings().indentAmount(0);
assertTrue(doc.html().contains(doc.select("title").outerHtml()));
assertTrue(doc.html().contains(doc.select("div").outerHtml()));
}
@Test public void testGetTextNodes() {
Document doc = Jsoup.parse("<p>One <span>Two</span> Three <br> Four</p>");
List<TextNode> textNodes = doc.select("p").first().textNodes();
assertEquals(3, textNodes.size());
assertEquals("One ", textNodes.get(0).text());
assertEquals(" Three ", textNodes.get(1).text());
assertEquals(" Four", textNodes.get(2).text());
assertEquals(0, doc.select("br").first().textNodes().size());
}
@Test public void testManipulateTextNodes() {
Document doc = Jsoup.parse("<p>One <span>Two</span> Three <br> Four</p>");
Element p = doc.select("p").first();
List<TextNode> textNodes = p.textNodes();
textNodes.get(1).text(" three-more ");
textNodes.get(2).splitText(3).text("-ur");
assertEquals("One Two three-more Fo-ur", p.text());
assertEquals("One three-more Fo-ur", p.ownText());
assertEquals(4, p.textNodes().size()); // grew because of split
}
@Test public void testGetDataNodes() {
Document doc = Jsoup.parse("<script>One Two</script> <style>Three Four</style> <p>Fix Six</p>");
Element script = doc.select("script").first();
Element style = doc.select("style").first();
Element p = doc.select("p").first();
List<DataNode> scriptData = script.dataNodes();
assertEquals(1, scriptData.size());
assertEquals("One Two", scriptData.get(0).getWholeData());
List<DataNode> styleData = style.dataNodes();
assertEquals(1, styleData.size());
assertEquals("Three Four", styleData.get(0).getWholeData());
List<DataNode> pData = p.dataNodes();
assertEquals(0, pData.size());
}
@Test public void elementIsNotASiblingOfItself() {
Document doc = Jsoup.parse("<div><p>One<p>Two<p>Three</div>");
Element p2 = doc.select("p").get(1);
assertEquals("Two", p2.text());
Elements els = p2.siblingElements();
assertEquals(2, els.size());
assertEquals("<p>One</p>", els.get(0).outerHtml());
assertEquals("<p>Three</p>", els.get(1).outerHtml());
}
@Test public void testChildThrowsIndexOutOfBoundsOnMissing() {
Document doc = Jsoup.parse("<div><p>One</p><p>Two</p></div>");
Element div = doc.select("div").first();
assertEquals(2, div.children().size());
assertEquals("One", div.child(0).text());
try {
div.child(3);
fail("Should throw index out of bounds");
} catch (IndexOutOfBoundsException e) {}
}
@Test
public void moveByAppend() {
// test for https://github.com/jhy/jsoup/issues/239
// can empty an element and append its children to another element
Document doc = Jsoup.parse("<div id=1>Text <p>One</p> Text <p>Two</p></div><div id=2></div>");
Element div1 = doc.select("div").get(0);
Element div2 = doc.select("div").get(1);
assertEquals(4, div1.childNodeSize());
List<Node> children = div1.childNodes();
assertEquals(4, children.size());
div2.insertChildren(0, children);
assertEquals(0, children.size()); // children is backed by div1.childNodes, moved, so should be 0 now
assertEquals(0, div1.childNodeSize());
assertEquals(4, div2.childNodeSize());
assertEquals("<div id=\"1\"></div>\n<div id=\"2\">\n Text \n <p>One</p> Text \n <p>Two</p>\n</div>",
doc.body().html());
}
@Test
public void insertChildrenArgumentValidation() {
Document doc = Jsoup.parse("<div id=1>Text <p>One</p> Text <p>Two</p></div><div id=2></div>");
Element div1 = doc.select("div").get(0);
Element div2 = doc.select("div").get(1);
List<Node> children = div1.childNodes();
try {
div2.insertChildren(6, children);
fail();
} catch (IllegalArgumentException e) {}
try {
div2.insertChildren(-5, children);
fail();
} catch (IllegalArgumentException e) {
}
try {
div2.insertChildren(0, null);
fail();
} catch (IllegalArgumentException e) {
}
}
@Test
public void insertChildrenAtPosition() {
Document doc = Jsoup.parse("<div id=1>Text1 <p>One</p> Text2 <p>Two</p></div><div id=2>Text3 <p>Three</p></div>");
Element div1 = doc.select("div").get(0);
Elements p1s = div1.select("p");
Element div2 = doc.select("div").get(1);
assertEquals(2, div2.childNodeSize());
div2.insertChildren(-1, p1s);
assertEquals(2, div1.childNodeSize()); // moved two out
assertEquals(4, div2.childNodeSize());
assertEquals(3, p1s.get(1).siblingIndex()); // should be last
List<Node> els = new ArrayList<Node>();
Element el1 = new Element(Tag.valueOf("span"), "").text("Span1");
Element el2 = new Element(Tag.valueOf("span"), "").text("Span2");
TextNode tn1 = new TextNode("Text4", "");
els.add(el1);
els.add(el2);
els.add(tn1);
assertNull(el1.parent());
div2.insertChildren(-2, els);
assertEquals(div2, el1.parent());
assertEquals(7, div2.childNodeSize());
assertEquals(3, el1.siblingIndex());
assertEquals(4, el2.siblingIndex());
assertEquals(5, tn1.siblingIndex());
}
@Test
public void insertChildrenAsCopy() {
Document doc = Jsoup.parse("<div id=1>Text <p>One</p> Text <p>Two</p></div><div id=2></div>");
Element div1 = doc.select("div").get(0);
Element div2 = doc.select("div").get(1);
Elements ps = doc.select("p").clone();
ps.first().text("One cloned");
div2.insertChildren(-1, ps);
assertEquals(4, div1.childNodeSize()); // not moved -- cloned
assertEquals(2, div2.childNodeSize());
assertEquals("<div id=\"1\">Text <p>One</p> Text <p>Two</p></div><div id=\"2\"><p>One cloned</p><p>Two</p></div>",
TextUtil.stripNewlines(doc.body().html()));
}
@Test
public void testCssPath() {
Document doc = Jsoup.parse("<div id=\"id1\">A</div><div>B</div><div class=\"c1 c2\">C</div>");
Element divA = doc.select("div").get(0);
Element divB = doc.select("div").get(1);
Element divC = doc.select("div").get(2);
assertEquals(divA.cssSelector(), "#id1");
assertEquals(divB.cssSelector(), "html > body > div:nth-child(2)");
assertEquals(divC.cssSelector(), "html > body > div.c1.c2");
assertTrue(divA == doc.select(divA.cssSelector()).first());
assertTrue(divB == doc.select(divB.cssSelector()).first());
assertTrue(divC == doc.select(divC.cssSelector()).first());
}
@Test
public void testClassNames() {
Document doc = Jsoup.parse("<div class=\"c1 c2\">C</div>");
Element div = doc.select("div").get(0);
assertEquals("c1 c2", div.className());
final Set<String> set1 = div.classNames();
final Object[] arr1 = set1.toArray();
assertTrue(arr1.length==2);
assertEquals("c1", arr1[0]);
assertEquals("c2", arr1[1]);
// Changes to the set should not be reflected in the Elements getters
set1.add("c3");
assertTrue(2==div.classNames().size());
assertEquals("c1 c2", div.className());
// Update the class names to a fresh set
final Set<String> newSet = new LinkedHashSet<String>(3);
newSet.addAll(set1);
newSet.add("c3");
div.classNames(newSet);
assertEquals("c1 c2 c3", div.className());
final Set<String> set2 = div.classNames();
final Object[] arr2 = set2.toArray();
assertTrue(arr2.length==3);
assertEquals("c1", arr2[0]);
assertEquals("c2", arr2[1]);
assertEquals("c3", arr2[2]);
}
@Test
public void testHashAndEquals() {
String doc1 = "<div id=1><p class=one>One</p><p class=one>One</p><p class=one>Two</p><p class=two>One</p></div>" +
"<div id=2><p class=one>One</p><p class=one>One</p><p class=one>Two</p><p class=two>One</p></div>";
Document doc = Jsoup.parse(doc1);
Elements els = doc.select("p");
/*
for (Element el : els) {
System.out.println(el.hashCode() + " - " + el.outerHtml());
}
0 1534787905 - <p class="one">One</p>
1 1534787905 - <p class="one">One</p>
2 1539683239 - <p class="one">Two</p>
3 1535455211 - <p class="two">One</p>
4 1534787905 - <p class="one">One</p>
5 1534787905 - <p class="one">One</p>
6 1539683239 - <p class="one">Two</p>
7 1535455211 - <p class="two">One</p>
*/
assertEquals(8, els.size());
Element e0 = els.get(0);
Element e1 = els.get(1);
Element e2 = els.get(2);
Element e3 = els.get(3);
Element e4 = els.get(4);
Element e5 = els.get(5);
Element e6 = els.get(6);
Element e7 = els.get(7);
assertEquals(e0, e1);
assertEquals(e0, e4);
assertEquals(e0, e5);
assertFalse(e0.equals(e2));
assertFalse(e0.equals(e3));
assertFalse(e0.equals(e6));
assertFalse(e0.equals(e7));
assertEquals(e0.hashCode(), e1.hashCode());
assertEquals(e0.hashCode(), e4.hashCode());
assertEquals(e0.hashCode(), e5.hashCode());
assertFalse(e0.hashCode() == (e2.hashCode()));
assertFalse(e0.hashCode() == (e3).hashCode());
assertFalse(e0.hashCode() == (e6).hashCode());
assertFalse(e0.hashCode() == (e7).hashCode());
}
} |
||
public void testExpand()
{
TextBuffer tb = new TextBuffer(new BufferRecycler());
char[] buf = tb.getCurrentSegment();
while (buf.length < 500 * 1000) {
char[] old = buf;
buf = tb.expandCurrentSegment();
if (old.length >= buf.length) {
fail("Expected buffer of "+old.length+" to expand, did not, length now "+buf.length);
}
}
} | com.fasterxml.jackson.core.util.TestTextBuffer::testExpand | src/test/java/com/fasterxml/jackson/core/util/TestTextBuffer.java | 78 | src/test/java/com/fasterxml/jackson/core/util/TestTextBuffer.java | testExpand | package com.fasterxml.jackson.core.util;
import com.fasterxml.jackson.core.util.BufferRecycler;
import com.fasterxml.jackson.core.util.TextBuffer;
public class TestTextBuffer
extends com.fasterxml.jackson.core.BaseTest
{
/**
* Trivially simple basic test to ensure all basic append
* methods work
*/
public void testSimple()
{
TextBuffer tb = new TextBuffer(new BufferRecycler());
tb.append('a');
tb.append(new char[] { 'X', 'b' }, 1, 1);
tb.append("c", 0, 1);
assertEquals(3, tb.contentsAsArray().length);
assertEquals("abc", tb.toString());
assertNotNull(tb.expandCurrentSegment());
}
public void testLonger()
{
TextBuffer tb = new TextBuffer(new BufferRecycler());
for (int i = 0; i < 2000; ++i) {
tb.append("abc", 0, 3);
}
String str = tb.contentsAsString();
assertEquals(6000, str.length());
assertEquals(6000, tb.contentsAsArray().length);
tb.resetWithShared(new char[] { 'a' }, 0, 1);
assertEquals(1, tb.toString().length());
}
public void testLongAppend()
{
final int len = TextBuffer.MAX_SEGMENT_LEN * 3 / 2;
StringBuilder sb = new StringBuilder(len);
for (int i = 0; i < len; ++i) {
sb.append('x');
}
final String STR = sb.toString();
final String EXP = "a" + STR + "c";
// ok: first test with String:
TextBuffer tb = new TextBuffer(new BufferRecycler());
tb.append('a');
tb.append(STR, 0, len);
tb.append('c');
assertEquals(len+2, tb.size());
assertEquals(EXP, tb.contentsAsString());
// then char[]
tb = new TextBuffer(new BufferRecycler());
tb.append('a');
tb.append(STR.toCharArray(), 0, len);
tb.append('c');
assertEquals(len+2, tb.size());
assertEquals(EXP, tb.contentsAsString());
}
// [Core#152]
public void testExpand()
{
TextBuffer tb = new TextBuffer(new BufferRecycler());
char[] buf = tb.getCurrentSegment();
while (buf.length < 500 * 1000) {
char[] old = buf;
buf = tb.expandCurrentSegment();
if (old.length >= buf.length) {
fail("Expected buffer of "+old.length+" to expand, did not, length now "+buf.length);
}
}
}
} | // You are a professional Java test case writer, please create a test case named `testExpand` for the issue `JacksonCore-152`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonCore-152
//
// ## Issue-Title:
// What is the maximum key length allowed?
//
// ## Issue-Description:
// I noticed that even in Jackson 2.4, if a JSON key is longer than 262144 bytes, ArrayIndexOutOfBoundsException is thrown from TextBuffer. Below is the stack trace:
//
//
//
// ```
// java.lang.ArrayIndexOutOfBoundsException
// at java.lang.System.arraycopy(Native Method)
// at com.fasterxml.jackson.core.util.TextBuffer.expandCurrentSegment(TextBuffer.java:604)
// at com.fasterxml.jackson.core.json.UTF8StreamJsonParser.addName(UTF8StreamJsonParser.java:2034)
// at com.fasterxml.jackson.core.json.UTF8StreamJsonParser.findName(UTF8StreamJsonParser.java:1928)
// at com.fasterxml.jackson.core.json.UTF8StreamJsonParser.parseLongFieldName(UTF8StreamJsonParser.java:1534)
// at com.fasterxml.jackson.core.json.UTF8StreamJsonParser.parseMediumFieldName(UTF8StreamJsonParser.java:1502)
// at com.fasterxml.jackson.core.json.UTF8StreamJsonParser._parseFieldName(UTF8StreamJsonParser.java:1437)
// at com.fasterxml.jackson.core.json.UTF8StreamJsonParser.nextToken(UTF8StreamJsonParser.java:668)
// ... <below are our code> ...
//
// ```
//
// Looking at TextBuffer.expandCurrentSegment(TextBuffer.java:604), once the length of \_currentSegment is increased to MAX\_SEGMENT\_LEN + 1 (262145) bytes, the newLen will stay at MAX\_SEGMENT\_LEN, which is smaller than len. Therefore System.arraycopy() will fail.
//
//
// I understand it is rare to have key larger than 262144 bytes, but it would be nice if
//
//
// * Jackson explicitly throw exception stating that key is too long.
// * Document that the maximum key length is 262144 bytes.
//
//
// OR
//
//
// * Update TextBuffer to support super long key.
//
//
// Thanks!
//
//
//
//
public void testExpand() {
| 78 | // [Core#152] | 4 | 66 | src/test/java/com/fasterxml/jackson/core/util/TestTextBuffer.java | src/test/java | ```markdown
## Issue-ID: JacksonCore-152
## Issue-Title:
What is the maximum key length allowed?
## Issue-Description:
I noticed that even in Jackson 2.4, if a JSON key is longer than 262144 bytes, ArrayIndexOutOfBoundsException is thrown from TextBuffer. Below is the stack trace:
```
java.lang.ArrayIndexOutOfBoundsException
at java.lang.System.arraycopy(Native Method)
at com.fasterxml.jackson.core.util.TextBuffer.expandCurrentSegment(TextBuffer.java:604)
at com.fasterxml.jackson.core.json.UTF8StreamJsonParser.addName(UTF8StreamJsonParser.java:2034)
at com.fasterxml.jackson.core.json.UTF8StreamJsonParser.findName(UTF8StreamJsonParser.java:1928)
at com.fasterxml.jackson.core.json.UTF8StreamJsonParser.parseLongFieldName(UTF8StreamJsonParser.java:1534)
at com.fasterxml.jackson.core.json.UTF8StreamJsonParser.parseMediumFieldName(UTF8StreamJsonParser.java:1502)
at com.fasterxml.jackson.core.json.UTF8StreamJsonParser._parseFieldName(UTF8StreamJsonParser.java:1437)
at com.fasterxml.jackson.core.json.UTF8StreamJsonParser.nextToken(UTF8StreamJsonParser.java:668)
... <below are our code> ...
```
Looking at TextBuffer.expandCurrentSegment(TextBuffer.java:604), once the length of \_currentSegment is increased to MAX\_SEGMENT\_LEN + 1 (262145) bytes, the newLen will stay at MAX\_SEGMENT\_LEN, which is smaller than len. Therefore System.arraycopy() will fail.
I understand it is rare to have key larger than 262144 bytes, but it would be nice if
* Jackson explicitly throw exception stating that key is too long.
* Document that the maximum key length is 262144 bytes.
OR
* Update TextBuffer to support super long key.
Thanks!
```
You are a professional Java test case writer, please create a test case named `testExpand` for the issue `JacksonCore-152`, utilizing the provided issue report information and the following function signature.
```java
public void testExpand() {
```
| 66 | [
"com.fasterxml.jackson.core.util.TextBuffer"
] | 9e9d7cb425aca518f8ed4d5ceb074cf2899b38ded5c6c0f273626afc51d5d252 | public void testExpand()
| // You are a professional Java test case writer, please create a test case named `testExpand` for the issue `JacksonCore-152`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonCore-152
//
// ## Issue-Title:
// What is the maximum key length allowed?
//
// ## Issue-Description:
// I noticed that even in Jackson 2.4, if a JSON key is longer than 262144 bytes, ArrayIndexOutOfBoundsException is thrown from TextBuffer. Below is the stack trace:
//
//
//
// ```
// java.lang.ArrayIndexOutOfBoundsException
// at java.lang.System.arraycopy(Native Method)
// at com.fasterxml.jackson.core.util.TextBuffer.expandCurrentSegment(TextBuffer.java:604)
// at com.fasterxml.jackson.core.json.UTF8StreamJsonParser.addName(UTF8StreamJsonParser.java:2034)
// at com.fasterxml.jackson.core.json.UTF8StreamJsonParser.findName(UTF8StreamJsonParser.java:1928)
// at com.fasterxml.jackson.core.json.UTF8StreamJsonParser.parseLongFieldName(UTF8StreamJsonParser.java:1534)
// at com.fasterxml.jackson.core.json.UTF8StreamJsonParser.parseMediumFieldName(UTF8StreamJsonParser.java:1502)
// at com.fasterxml.jackson.core.json.UTF8StreamJsonParser._parseFieldName(UTF8StreamJsonParser.java:1437)
// at com.fasterxml.jackson.core.json.UTF8StreamJsonParser.nextToken(UTF8StreamJsonParser.java:668)
// ... <below are our code> ...
//
// ```
//
// Looking at TextBuffer.expandCurrentSegment(TextBuffer.java:604), once the length of \_currentSegment is increased to MAX\_SEGMENT\_LEN + 1 (262145) bytes, the newLen will stay at MAX\_SEGMENT\_LEN, which is smaller than len. Therefore System.arraycopy() will fail.
//
//
// I understand it is rare to have key larger than 262144 bytes, but it would be nice if
//
//
// * Jackson explicitly throw exception stating that key is too long.
// * Document that the maximum key length is 262144 bytes.
//
//
// OR
//
//
// * Update TextBuffer to support super long key.
//
//
// Thanks!
//
//
//
//
| JacksonCore | package com.fasterxml.jackson.core.util;
import com.fasterxml.jackson.core.util.BufferRecycler;
import com.fasterxml.jackson.core.util.TextBuffer;
public class TestTextBuffer
extends com.fasterxml.jackson.core.BaseTest
{
/**
* Trivially simple basic test to ensure all basic append
* methods work
*/
public void testSimple()
{
TextBuffer tb = new TextBuffer(new BufferRecycler());
tb.append('a');
tb.append(new char[] { 'X', 'b' }, 1, 1);
tb.append("c", 0, 1);
assertEquals(3, tb.contentsAsArray().length);
assertEquals("abc", tb.toString());
assertNotNull(tb.expandCurrentSegment());
}
public void testLonger()
{
TextBuffer tb = new TextBuffer(new BufferRecycler());
for (int i = 0; i < 2000; ++i) {
tb.append("abc", 0, 3);
}
String str = tb.contentsAsString();
assertEquals(6000, str.length());
assertEquals(6000, tb.contentsAsArray().length);
tb.resetWithShared(new char[] { 'a' }, 0, 1);
assertEquals(1, tb.toString().length());
}
public void testLongAppend()
{
final int len = TextBuffer.MAX_SEGMENT_LEN * 3 / 2;
StringBuilder sb = new StringBuilder(len);
for (int i = 0; i < len; ++i) {
sb.append('x');
}
final String STR = sb.toString();
final String EXP = "a" + STR + "c";
// ok: first test with String:
TextBuffer tb = new TextBuffer(new BufferRecycler());
tb.append('a');
tb.append(STR, 0, len);
tb.append('c');
assertEquals(len+2, tb.size());
assertEquals(EXP, tb.contentsAsString());
// then char[]
tb = new TextBuffer(new BufferRecycler());
tb.append('a');
tb.append(STR.toCharArray(), 0, len);
tb.append('c');
assertEquals(len+2, tb.size());
assertEquals(EXP, tb.contentsAsString());
}
// [Core#152]
public void testExpand()
{
TextBuffer tb = new TextBuffer(new BufferRecycler());
char[] buf = tb.getCurrentSegment();
while (buf.length < 500 * 1000) {
char[] old = buf;
buf = tb.expandCurrentSegment();
if (old.length >= buf.length) {
fail("Expected buffer of "+old.length+" to expand, did not, length now "+buf.length);
}
}
}
} |
|
@Test
public void shouldWorkFineWhenGivenArgIsNull() {
//when
Integer[] suspicious = tool.getSuspiciouslyNotMatchingArgsIndexes((List) Arrays.asList(new Equals(20)), new Object[] {null});
//then
assertEquals(0, suspicious.length);
} | org.mockito.internal.verification.argumentmatching.ArgumentMatchingToolTest::shouldWorkFineWhenGivenArgIsNull | test/org/mockito/internal/verification/argumentmatching/ArgumentMatchingToolTest.java | 85 | test/org/mockito/internal/verification/argumentmatching/ArgumentMatchingToolTest.java | shouldWorkFineWhenGivenArgIsNull | /*
* Copyright (c) 2007 Mockito contributors
* This program is made available under the terms of the MIT License.
*/
package org.mockito.internal.verification.argumentmatching;
import java.util.Arrays;
import java.util.List;
import org.hamcrest.BaseMatcher;
import org.hamcrest.Description;
import org.hamcrest.Matcher;
import org.junit.Test;
import org.mockito.internal.matchers.Equals;
import org.mockitoutil.TestBase;
@SuppressWarnings("unchecked")
public class ArgumentMatchingToolTest extends TestBase {
private ArgumentMatchingTool tool = new ArgumentMatchingTool();
@Test
public void shouldNotFindAnySuspiciousMatchersWhenNumberOfArgumentsDoesntMatch() {
//given
List<Matcher> matchers = (List) Arrays.asList(new Equals(1));
//when
Integer[] suspicious = tool.getSuspiciouslyNotMatchingArgsIndexes(matchers, new Object[] {10, 20});
//then
assertEquals(0, suspicious.length);
}
@Test
public void shouldNotFindAnySuspiciousMatchersWhenArgumentsMatch() {
//given
List<Matcher> matchers = (List) Arrays.asList(new Equals(10), new Equals(20));
//when
Integer[] suspicious = tool.getSuspiciouslyNotMatchingArgsIndexes(matchers, new Object[] {10, 20});
//then
assertEquals(0, suspicious.length);
}
@Test
public void shouldFindSuspiciousMatchers() {
//given
Equals matcherInt20 = new Equals(20);
Long longPretendingAnInt = new Long(20);
//when
List<Matcher> matchers = (List) Arrays.asList(new Equals(10), matcherInt20);
Integer[] suspicious = tool.getSuspiciouslyNotMatchingArgsIndexes(matchers, new Object[] {10, longPretendingAnInt});
//then
assertEquals(1, suspicious.length);
assertEquals(new Integer(1), suspicious[0]);
}
@Test
public void shouldNotFindSuspiciousMatchersWhenTypesAreTheSame() {
//given
Equals matcherWithBadDescription = new Equals(20) {
public void describeTo(Description desc) {
//let's pretend we have the same description as the toString() of the argument
desc.appendText("10");
}
};
Integer argument = 10;
//when
Integer[] suspicious = tool.getSuspiciouslyNotMatchingArgsIndexes((List) Arrays.asList(matcherWithBadDescription), new Object[] {argument});
//then
assertEquals(0, suspicious.length);
}
@Test
public void shouldWorkFineWhenGivenArgIsNull() {
//when
Integer[] suspicious = tool.getSuspiciouslyNotMatchingArgsIndexes((List) Arrays.asList(new Equals(20)), new Object[] {null});
//then
assertEquals(0, suspicious.length);
}
@Test
public void shouldUseMatchersSafely() {
//given
List<Matcher> matchers = (List) Arrays.asList(new BaseMatcher() {
public boolean matches(Object item) {
throw new ClassCastException("nasty matcher");
}
public void describeTo(Description description) {
}});
//when
Integer[] suspicious = tool.getSuspiciouslyNotMatchingArgsIndexes(matchers, new Object[] {10});
//then
assertEquals(0, suspicious.length);
}
} | // You are a professional Java test case writer, please create a test case named `shouldWorkFineWhenGivenArgIsNull` for the issue `Mockito-79`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Mockito-79
//
// ## Issue-Title:
// Generate change list separated by types using labels
//
// ## Issue-Description:
// [](https://coveralls.io/builds/1188420)
//
//
// Changes Unknown when pulling **[47a7016](https://github.com/mockito/mockito/commit/47a701638ebf4673ed0f1c8680d4d8f9f79c4f6e) on szpak:topic/releaseLabels** into *\* on mockito:master*\*.
//
//
//
//
@Test
public void shouldWorkFineWhenGivenArgIsNull() {
| 85 | 38 | 78 | test/org/mockito/internal/verification/argumentmatching/ArgumentMatchingToolTest.java | test | ```markdown
## Issue-ID: Mockito-79
## Issue-Title:
Generate change list separated by types using labels
## Issue-Description:
[](https://coveralls.io/builds/1188420)
Changes Unknown when pulling **[47a7016](https://github.com/mockito/mockito/commit/47a701638ebf4673ed0f1c8680d4d8f9f79c4f6e) on szpak:topic/releaseLabels** into *\* on mockito:master*\*.
```
You are a professional Java test case writer, please create a test case named `shouldWorkFineWhenGivenArgIsNull` for the issue `Mockito-79`, utilizing the provided issue report information and the following function signature.
```java
@Test
public void shouldWorkFineWhenGivenArgIsNull() {
```
| 78 | [
"org.mockito.internal.verification.argumentmatching.ArgumentMatchingTool"
] | 9f16470a1624d256c6f8b3481c8e850ee5de38c8b6634ab83f1bf028da23e824 | @Test
public void shouldWorkFineWhenGivenArgIsNull() | // You are a professional Java test case writer, please create a test case named `shouldWorkFineWhenGivenArgIsNull` for the issue `Mockito-79`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Mockito-79
//
// ## Issue-Title:
// Generate change list separated by types using labels
//
// ## Issue-Description:
// [](https://coveralls.io/builds/1188420)
//
//
// Changes Unknown when pulling **[47a7016](https://github.com/mockito/mockito/commit/47a701638ebf4673ed0f1c8680d4d8f9f79c4f6e) on szpak:topic/releaseLabels** into *\* on mockito:master*\*.
//
//
//
//
| Mockito | /*
* Copyright (c) 2007 Mockito contributors
* This program is made available under the terms of the MIT License.
*/
package org.mockito.internal.verification.argumentmatching;
import java.util.Arrays;
import java.util.List;
import org.hamcrest.BaseMatcher;
import org.hamcrest.Description;
import org.hamcrest.Matcher;
import org.junit.Test;
import org.mockito.internal.matchers.Equals;
import org.mockitoutil.TestBase;
@SuppressWarnings("unchecked")
public class ArgumentMatchingToolTest extends TestBase {
private ArgumentMatchingTool tool = new ArgumentMatchingTool();
@Test
public void shouldNotFindAnySuspiciousMatchersWhenNumberOfArgumentsDoesntMatch() {
//given
List<Matcher> matchers = (List) Arrays.asList(new Equals(1));
//when
Integer[] suspicious = tool.getSuspiciouslyNotMatchingArgsIndexes(matchers, new Object[] {10, 20});
//then
assertEquals(0, suspicious.length);
}
@Test
public void shouldNotFindAnySuspiciousMatchersWhenArgumentsMatch() {
//given
List<Matcher> matchers = (List) Arrays.asList(new Equals(10), new Equals(20));
//when
Integer[] suspicious = tool.getSuspiciouslyNotMatchingArgsIndexes(matchers, new Object[] {10, 20});
//then
assertEquals(0, suspicious.length);
}
@Test
public void shouldFindSuspiciousMatchers() {
//given
Equals matcherInt20 = new Equals(20);
Long longPretendingAnInt = new Long(20);
//when
List<Matcher> matchers = (List) Arrays.asList(new Equals(10), matcherInt20);
Integer[] suspicious = tool.getSuspiciouslyNotMatchingArgsIndexes(matchers, new Object[] {10, longPretendingAnInt});
//then
assertEquals(1, suspicious.length);
assertEquals(new Integer(1), suspicious[0]);
}
@Test
public void shouldNotFindSuspiciousMatchersWhenTypesAreTheSame() {
//given
Equals matcherWithBadDescription = new Equals(20) {
public void describeTo(Description desc) {
//let's pretend we have the same description as the toString() of the argument
desc.appendText("10");
}
};
Integer argument = 10;
//when
Integer[] suspicious = tool.getSuspiciouslyNotMatchingArgsIndexes((List) Arrays.asList(matcherWithBadDescription), new Object[] {argument});
//then
assertEquals(0, suspicious.length);
}
@Test
public void shouldWorkFineWhenGivenArgIsNull() {
//when
Integer[] suspicious = tool.getSuspiciouslyNotMatchingArgsIndexes((List) Arrays.asList(new Equals(20)), new Object[] {null});
//then
assertEquals(0, suspicious.length);
}
@Test
public void shouldUseMatchersSafely() {
//given
List<Matcher> matchers = (List) Arrays.asList(new BaseMatcher() {
public boolean matches(Object item) {
throw new ClassCastException("nasty matcher");
}
public void describeTo(Description description) {
}});
//when
Integer[] suspicious = tool.getSuspiciouslyNotMatchingArgsIndexes(matchers, new Object[] {10});
//then
assertEquals(0, suspicious.length);
}
} |
||
public void testZero() {
assertPrint("var x ='\\0';", "var x=\"\\000\"");
assertPrint("var x ='\\x00';", "var x=\"\\000\"");
assertPrint("var x ='\\u0000';", "var x=\"\\000\"");
assertPrint("var x ='\\u00003';", "var x=\"\\0003\"");
} | com.google.javascript.jscomp.CodePrinterTest::testZero | test/com/google/javascript/jscomp/CodePrinterTest.java | 1,234 | test/com/google/javascript/jscomp/CodePrinterTest.java | testZero | /*
* Copyright 2004 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import com.google.javascript.jscomp.CompilerOptions.LanguageMode;
import com.google.javascript.rhino.Node;
import com.google.javascript.rhino.Token;
import junit.framework.TestCase;
public class CodePrinterTest extends TestCase {
static Node parse(String js) {
return parse(js, false);
}
static Node parse(String js, boolean checkTypes) {
Compiler compiler = new Compiler();
CompilerOptions options = new CompilerOptions();
// Allow getters and setters.
options.setLanguageIn(LanguageMode.ECMASCRIPT5);
compiler.initOptions(options);
Node n = compiler.parseTestCode(js);
if (checkTypes) {
DefaultPassConfig passConfig = new DefaultPassConfig(null);
CompilerPass typeResolver = passConfig.resolveTypes.create(compiler);
Node externs = new Node(Token.SCRIPT);
externs.setIsSyntheticBlock(true);
Node externAndJsRoot = new Node(Token.BLOCK, externs, n);
externAndJsRoot.setIsSyntheticBlock(true);
typeResolver.process(externs, n);
CompilerPass inferTypes = passConfig.inferTypes.create(compiler);
inferTypes.process(externs, n);
}
checkUnexpectedErrorsOrWarnings(compiler, 0);
return n;
}
private static void checkUnexpectedErrorsOrWarnings(
Compiler compiler, int expected) {
int actual = compiler.getErrors().length + compiler.getWarnings().length;
if (actual != expected) {
String msg = "";
for (JSError err : compiler.getErrors()) {
msg += "Error:" + err.toString() + "\n";
}
for (JSError err : compiler.getWarnings()) {
msg += "Warning:" + err.toString() + "\n";
}
assertEquals("Unexpected warnings or errors.\n " + msg, expected, actual);
}
}
String parsePrint(String js, boolean prettyprint, int lineThreshold) {
return new CodePrinter.Builder(parse(js)).setPrettyPrint(prettyprint)
.setLineLengthThreshold(lineThreshold).build();
}
String parsePrint(String js, boolean prettyprint, boolean lineBreak,
int lineThreshold) {
return new CodePrinter.Builder(parse(js)).setPrettyPrint(prettyprint)
.setLineLengthThreshold(lineThreshold).setLineBreak(lineBreak).build();
}
String parsePrint(String js, boolean prettyprint, boolean lineBreak,
int lineThreshold, boolean outputTypes) {
return new CodePrinter.Builder(parse(js, true)).setPrettyPrint(prettyprint)
.setOutputTypes(outputTypes)
.setLineLengthThreshold(lineThreshold).setLineBreak(lineBreak)
.build();
}
String parsePrint(String js, boolean prettyprint, boolean lineBreak,
int lineThreshold, boolean outputTypes,
boolean tagAsStrict) {
return new CodePrinter.Builder(parse(js, true)).setPrettyPrint(prettyprint)
.setOutputTypes(outputTypes)
.setLineLengthThreshold(lineThreshold).setLineBreak(lineBreak)
.setTagAsStrict(tagAsStrict)
.build();
}
String printNode(Node n) {
return new CodePrinter.Builder(n).setLineLengthThreshold(
CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD).build();
}
void assertPrintNode(String expectedJs, Node ast) {
assertEquals(expectedJs, printNode(ast));
}
public void testPrint() {
assertPrint("10 + a + b", "10+a+b");
assertPrint("10 + (30*50)", "10+30*50");
assertPrint("with(x) { x + 3; }", "with(x)x+3");
assertPrint("\"aa'a\"", "\"aa'a\"");
assertPrint("\"aa\\\"a\"", "'aa\"a'");
assertPrint("function foo()\n{return 10;}", "function foo(){return 10}");
assertPrint("a instanceof b", "a instanceof b");
assertPrint("typeof(a)", "typeof a");
assertPrint(
"var foo = x ? { a : 1 } : {a: 3, b:4, \"default\": 5, \"foo-bar\": 6}",
"var foo=x?{a:1}:{a:3,b:4,\"default\":5,\"foo-bar\":6}");
// Safari: needs ';' at the end of a throw statement
assertPrint("function foo(){throw 'error';}",
"function foo(){throw\"error\";}");
// Safari 3 needs a "{" around a single function
assertPrint("if (true) function foo(){return}",
"if(true){function foo(){return}}");
assertPrint("var x = 10; { var y = 20; }", "var x=10;var y=20");
assertPrint("while (x-- > 0);", "while(x-- >0);");
assertPrint("x-- >> 1", "x-- >>1");
assertPrint("(function () {})(); ",
"(function(){})()");
// Associativity
assertPrint("var a,b,c,d;a || (b&& c) && (a || d)",
"var a,b,c,d;a||b&&c&&(a||d)");
assertPrint("var a,b,c; a || (b || c); a * (b * c); a | (b | c)",
"var a,b,c;a||b||c;a*b*c;a|b|c");
assertPrint("var a,b,c; a / b / c;a / (b / c); a - (b - c);",
"var a,b,c;a/b/c;a/(b/c);a-(b-c)");
assertPrint("var a,b; a = b = 3;",
"var a,b;a=b=3");
assertPrint("var a,b,c,d; a = (b = c = (d = 3));",
"var a,b,c,d;a=b=c=d=3");
assertPrint("var a,b,c; a += (b = c += 3);",
"var a,b,c;a+=b=c+=3");
assertPrint("var a,b,c; a *= (b -= c);",
"var a,b,c;a*=b-=c");
// Break scripts
assertPrint("'<script>'", "\"<script>\"");
assertPrint("'</script>'", "\"<\\/script>\"");
assertPrint("\"</script> </SCRIPT>\"", "\"<\\/script> <\\/SCRIPT>\"");
assertPrint("'-->'", "\"--\\>\"");
assertPrint("']]>'", "\"]]\\>\"");
assertPrint("' --></script>'", "\" --\\><\\/script>\"");
assertPrint("/--> <\\/script>/g", "/--\\> <\\/script>/g");
// Break HTML start comments. Certain versions of Webkit
// begin an HTML comment when they see this.
assertPrint("'<!-- I am a string -->'", "\"<\\!-- I am a string --\\>\"");
// Precedence
assertPrint("a ? delete b[0] : 3", "a?delete b[0]:3");
assertPrint("(delete a[0])/10", "delete a[0]/10");
// optional '()' for new
// simple new
assertPrint("new A", "new A");
assertPrint("new A()", "new A");
assertPrint("new A('x')", "new A(\"x\")");
// calling instance method directly after new
assertPrint("new A().a()", "(new A).a()");
assertPrint("(new A).a()", "(new A).a()");
// this case should be fixed
assertPrint("new A('y').a()", "(new A(\"y\")).a()");
// internal class
assertPrint("new A.B", "new A.B");
assertPrint("new A.B()", "new A.B");
assertPrint("new A.B('z')", "new A.B(\"z\")");
// calling instance method directly after new internal class
assertPrint("(new A.B).a()", "(new A.B).a()");
assertPrint("new A.B().a()", "(new A.B).a()");
// this case should be fixed
assertPrint("new A.B('w').a()", "(new A.B(\"w\")).a()");
// Operators: make sure we don't convert binary + and unary + into ++
assertPrint("x + +y", "x+ +y");
assertPrint("x - (-y)", "x- -y");
assertPrint("x++ +y", "x++ +y");
assertPrint("x-- -y", "x-- -y");
assertPrint("x++ -y", "x++-y");
// Label
assertPrint("foo:for(;;){break foo;}", "foo:for(;;)break foo");
assertPrint("foo:while(1){continue foo;}", "foo:while(1)continue foo");
// Object literals.
assertPrint("({})", "({})");
assertPrint("var x = {};", "var x={}");
assertPrint("({}).x", "({}).x");
assertPrint("({})['x']", "({})[\"x\"]");
assertPrint("({}) instanceof Object", "({})instanceof Object");
assertPrint("({}) || 1", "({})||1");
assertPrint("1 || ({})", "1||{}");
assertPrint("({}) ? 1 : 2", "({})?1:2");
assertPrint("0 ? ({}) : 2", "0?{}:2");
assertPrint("0 ? 1 : ({})", "0?1:{}");
assertPrint("typeof ({})", "typeof{}");
assertPrint("f({})", "f({})");
// Anonymous function expressions.
assertPrint("(function(){})", "(function(){})");
assertPrint("(function(){})()", "(function(){})()");
assertPrint("(function(){})instanceof Object",
"(function(){})instanceof Object");
assertPrint("(function(){}).bind().call()",
"(function(){}).bind().call()");
assertPrint("var x = function() { };", "var x=function(){}");
assertPrint("var x = function() { }();", "var x=function(){}()");
assertPrint("(function() {}), 2", "(function(){}),2");
// Name functions expression.
assertPrint("(function f(){})", "(function f(){})");
// Function declaration.
assertPrint("function f(){}", "function f(){}");
// Make sure we don't treat non-latin character escapes as raw strings.
assertPrint("({ 'a': 4, '\\u0100': 4 })", "({\"a\":4,\"\\u0100\":4})");
assertPrint("({ a: 4, '\\u0100': 4 })", "({a:4,\"\\u0100\":4})");
// Test if statement and for statements with single statements in body.
assertPrint("if (true) { alert();}", "if(true)alert()");
assertPrint("if (false) {} else {alert(\"a\");}",
"if(false);else alert(\"a\")");
assertPrint("for(;;) { alert();};", "for(;;)alert()");
assertPrint("do { alert(); } while(true);",
"do alert();while(true)");
assertPrint("myLabel: { alert();}",
"myLabel:alert()");
assertPrint("myLabel: for(;;) continue myLabel;",
"myLabel:for(;;)continue myLabel");
// Test nested var statement
assertPrint("if (true) var x; x = 4;", "if(true)var x;x=4");
// Non-latin identifier. Make sure we keep them escaped.
assertPrint("\\u00fb", "\\u00fb");
assertPrint("\\u00fa=1", "\\u00fa=1");
assertPrint("function \\u00f9(){}", "function \\u00f9(){}");
assertPrint("x.\\u00f8", "x.\\u00f8");
assertPrint("x.\\u00f8", "x.\\u00f8");
assertPrint("abc\\u4e00\\u4e01jkl", "abc\\u4e00\\u4e01jkl");
// Test the right-associative unary operators for spurious parens
assertPrint("! ! true", "!!true");
assertPrint("!(!(true))", "!!true");
assertPrint("typeof(void(0))", "typeof void 0");
assertPrint("typeof(void(!0))", "typeof void!0");
assertPrint("+ - + + - + 3", "+-+ +-+3"); // chained unary plus/minus
assertPrint("+(--x)", "+--x");
assertPrint("-(++x)", "-++x");
// needs a space to prevent an ambiguous parse
assertPrint("-(--x)", "- --x");
assertPrint("!(~~5)", "!~~5");
assertPrint("~(a/b)", "~(a/b)");
// Preserve parens to overcome greedy binding of NEW
assertPrint("new (foo.bar()).factory(baz)", "new (foo.bar().factory)(baz)");
assertPrint("new (bar()).factory(baz)", "new (bar().factory)(baz)");
assertPrint("new (new foobar(x)).factory(baz)",
"new (new foobar(x)).factory(baz)");
// Make sure that HOOK is right associative
assertPrint("a ? b : (c ? d : e)", "a?b:c?d:e");
assertPrint("a ? (b ? c : d) : e", "a?b?c:d:e");
assertPrint("(a ? b : c) ? d : e", "(a?b:c)?d:e");
// Test nested ifs
assertPrint("if (x) if (y); else;", "if(x)if(y);else;");
// Test comma.
assertPrint("a,b,c", "a,b,c");
assertPrint("(a,b),c", "a,b,c");
assertPrint("a,(b,c)", "a,b,c");
assertPrint("x=a,b,c", "x=a,b,c");
assertPrint("x=(a,b),c", "x=(a,b),c");
assertPrint("x=a,(b,c)", "x=a,b,c");
assertPrint("x=a,y=b,z=c", "x=a,y=b,z=c");
assertPrint("x=(a,y=b,z=c)", "x=(a,y=b,z=c)");
assertPrint("x=[a,b,c,d]", "x=[a,b,c,d]");
assertPrint("x=[(a,b,c),d]", "x=[(a,b,c),d]");
assertPrint("x=[(a,(b,c)),d]", "x=[(a,b,c),d]");
assertPrint("x=[a,(b,c,d)]", "x=[a,(b,c,d)]");
assertPrint("var x=(a,b)", "var x=(a,b)");
assertPrint("var x=a,b,c", "var x=a,b,c");
assertPrint("var x=(a,b),c", "var x=(a,b),c");
assertPrint("var x=a,b=(c,d)", "var x=a,b=(c,d)");
assertPrint("foo(a,b,c,d)", "foo(a,b,c,d)");
assertPrint("foo((a,b,c),d)", "foo((a,b,c),d)");
assertPrint("foo((a,(b,c)),d)", "foo((a,b,c),d)");
assertPrint("f(a+b,(c,d,(e,f,g)))", "f(a+b,(c,d,e,f,g))");
assertPrint("({}) , 1 , 2", "({}),1,2");
assertPrint("({}) , {} , {}", "({}),{},{}");
// EMPTY nodes
assertPrint("if (x){}", "if(x);");
assertPrint("if(x);", "if(x);");
assertPrint("if(x)if(y);", "if(x)if(y);");
assertPrint("if(x){if(y);}", "if(x)if(y);");
assertPrint("if(x){if(y){};;;}", "if(x)if(y);");
assertPrint("if(x){;;function y(){};;}", "if(x){function y(){}}");
}
public void testPrintArray() {
assertPrint("[void 0, void 0]", "[void 0,void 0]");
assertPrint("[undefined, undefined]", "[undefined,undefined]");
assertPrint("[ , , , undefined]", "[,,,undefined]");
assertPrint("[ , , , 0]", "[,,,0]");
}
public void testHook() {
assertPrint("a ? b = 1 : c = 2", "a?b=1:c=2");
assertPrint("x = a ? b = 1 : c = 2", "x=a?b=1:c=2");
assertPrint("(x = a) ? b = 1 : c = 2", "(x=a)?b=1:c=2");
assertPrint("x, a ? b = 1 : c = 2", "x,a?b=1:c=2");
assertPrint("x, (a ? b = 1 : c = 2)", "x,a?b=1:c=2");
assertPrint("(x, a) ? b = 1 : c = 2", "(x,a)?b=1:c=2");
assertPrint("a ? (x, b) : c = 2", "a?(x,b):c=2");
assertPrint("a ? b = 1 : (x,c)", "a?b=1:(x,c)");
assertPrint("a ? b = 1 : c = 2 + x", "a?b=1:c=2+x");
assertPrint("(a ? b = 1 : c = 2) + x", "(a?b=1:c=2)+x");
assertPrint("a ? b = 1 : (c = 2) + x", "a?b=1:(c=2)+x");
assertPrint("a ? (b?1:2) : 3", "a?b?1:2:3");
}
public void testPrintInOperatorInForLoop() {
// Check for in expression in for's init expression.
// Check alone, with + (higher precedence), with ?: (lower precedence),
// and with conditional.
assertPrint("var a={}; for (var i = (\"length\" in a); i;) {}",
"var a={};for(var i=(\"length\"in a);i;);");
assertPrint("var a={}; for (var i = (\"length\" in a) ? 0 : 1; i;) {}",
"var a={};for(var i=(\"length\"in a)?0:1;i;);");
assertPrint("var a={}; for (var i = (\"length\" in a) + 1; i;) {}",
"var a={};for(var i=(\"length\"in a)+1;i;);");
assertPrint("var a={};for (var i = (\"length\" in a|| \"size\" in a);;);",
"var a={};for(var i=(\"length\"in a)||(\"size\"in a);;);");
assertPrint("var a={};for (var i = a || a || (\"size\" in a);;);",
"var a={};for(var i=a||a||(\"size\"in a);;);");
// Test works with unary operators and calls.
assertPrint("var a={}; for (var i = -(\"length\" in a); i;) {}",
"var a={};for(var i=-(\"length\"in a);i;);");
assertPrint("var a={};function b_(p){ return p;};" +
"for(var i=1,j=b_(\"length\" in a);;) {}",
"var a={};function b_(p){return p}" +
"for(var i=1,j=b_(\"length\"in a);;);");
// Test we correctly handle an in operator in the test clause.
assertPrint("var a={}; for (;(\"length\" in a);) {}",
"var a={};for(;\"length\"in a;);");
}
public void testLiteralProperty() {
assertPrint("(64).toString()", "(64).toString()");
}
private void assertPrint(String js, String expected) {
parse(expected); // validate the expected string is valid js
assertEquals(expected,
parsePrint(js, false, CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD));
}
// Make sure that the code generator doesn't associate an
// else clause with the wrong if clause.
public void testAmbiguousElseClauses() {
assertPrintNode("if(x)if(y);else;",
new Node(Token.IF,
Node.newString(Token.NAME, "x"),
new Node(Token.BLOCK,
new Node(Token.IF,
Node.newString(Token.NAME, "y"),
new Node(Token.BLOCK),
// ELSE clause for the inner if
new Node(Token.BLOCK)))));
assertPrintNode("if(x){if(y);}else;",
new Node(Token.IF,
Node.newString(Token.NAME, "x"),
new Node(Token.BLOCK,
new Node(Token.IF,
Node.newString(Token.NAME, "y"),
new Node(Token.BLOCK))),
// ELSE clause for the outer if
new Node(Token.BLOCK)));
assertPrintNode("if(x)if(y);else{if(z);}else;",
new Node(Token.IF,
Node.newString(Token.NAME, "x"),
new Node(Token.BLOCK,
new Node(Token.IF,
Node.newString(Token.NAME, "y"),
new Node(Token.BLOCK),
new Node(Token.BLOCK,
new Node(Token.IF,
Node.newString(Token.NAME, "z"),
new Node(Token.BLOCK))))),
// ELSE clause for the outermost if
new Node(Token.BLOCK)));
}
public void testLineBreak() {
// line break after function if in a statement context
assertLineBreak("function a() {}\n" +
"function b() {}",
"function a(){}\n" +
"function b(){}\n");
// line break after ; after a function
assertLineBreak("var a = {};\n" +
"a.foo = function () {}\n" +
"function b() {}",
"var a={};a.foo=function(){};\n" +
"function b(){}\n");
// break after comma after a function
assertLineBreak("var a = {\n" +
" b: function() {},\n" +
" c: function() {}\n" +
"};\n" +
"alert(a);",
"var a={b:function(){},\n" +
"c:function(){}};\n" +
"alert(a)");
}
private void assertLineBreak(String js, String expected) {
assertEquals(expected,
parsePrint(js, false, true,
CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD));
}
public void testPrettyPrinter() {
// Ensure that the pretty printer inserts line breaks at appropriate
// places.
assertPrettyPrint("(function(){})();","(function() {\n})();\n");
assertPrettyPrint("var a = (function() {});alert(a);",
"var a = function() {\n};\nalert(a);\n");
// Check we correctly handle putting brackets around all if clauses so
// we can put breakpoints inside statements.
assertPrettyPrint("if (1) {}",
"if(1) {\n" +
"}\n");
assertPrettyPrint("if (1) {alert(\"\");}",
"if(1) {\n" +
" alert(\"\")\n" +
"}\n");
assertPrettyPrint("if (1)alert(\"\");",
"if(1) {\n" +
" alert(\"\")\n" +
"}\n");
assertPrettyPrint("if (1) {alert();alert();}",
"if(1) {\n" +
" alert();\n" +
" alert()\n" +
"}\n");
// Don't add blocks if they weren't there already.
assertPrettyPrint("label: alert();",
"label:alert();\n");
// But if statements and loops get blocks automagically.
assertPrettyPrint("if (1) alert();",
"if(1) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint("for (;;) alert();",
"for(;;) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint("while (1) alert();",
"while(1) {\n" +
" alert()\n" +
"}\n");
// Do we put else clauses in blocks?
assertPrettyPrint("if (1) {} else {alert(a);}",
"if(1) {\n" +
"}else {\n alert(a)\n}\n");
// Do we add blocks to else clauses?
assertPrettyPrint("if (1) alert(a); else alert(b);",
"if(1) {\n" +
" alert(a)\n" +
"}else {\n" +
" alert(b)\n" +
"}\n");
// Do we put for bodies in blocks?
assertPrettyPrint("for(;;) { alert();}",
"for(;;) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint("for(;;) {}",
"for(;;) {\n" +
"}\n");
assertPrettyPrint("for(;;) { alert(); alert(); }",
"for(;;) {\n" +
" alert();\n" +
" alert()\n" +
"}\n");
// How about do loops?
assertPrettyPrint("do { alert(); } while(true);",
"do {\n" +
" alert()\n" +
"}while(true);\n");
// label?
assertPrettyPrint("myLabel: { alert();}",
"myLabel: {\n" +
" alert()\n" +
"}\n");
// Don't move the label on a loop, because then break {label} and
// continue {label} won't work.
assertPrettyPrint("myLabel: for(;;) continue myLabel;",
"myLabel:for(;;) {\n" +
" continue myLabel\n" +
"}\n");
assertPrettyPrint("var a;", "var a;\n");
}
public void testPrettyPrinter2() {
assertPrettyPrint(
"if(true) f();",
"if(true) {\n" +
" f()\n" +
"}\n");
assertPrettyPrint(
"if (true) { f() } else { g() }",
"if(true) {\n" +
" f()\n" +
"}else {\n" +
" g()\n" +
"}\n");
assertPrettyPrint(
"if(true) f(); for(;;) g();",
"if(true) {\n" +
" f()\n" +
"}\n" +
"for(;;) {\n" +
" g()\n" +
"}\n");
}
public void testPrettyPrinter3() {
assertPrettyPrint(
"try {} catch(e) {}if (1) {alert();alert();}",
"try {\n" +
"}catch(e) {\n" +
"}\n" +
"if(1) {\n" +
" alert();\n" +
" alert()\n" +
"}\n");
assertPrettyPrint(
"try {} finally {}if (1) {alert();alert();}",
"try {\n" +
"}finally {\n" +
"}\n" +
"if(1) {\n" +
" alert();\n" +
" alert()\n" +
"}\n");
assertPrettyPrint(
"try {} catch(e) {} finally {} if (1) {alert();alert();}",
"try {\n" +
"}catch(e) {\n" +
"}finally {\n" +
"}\n" +
"if(1) {\n" +
" alert();\n" +
" alert()\n" +
"}\n");
}
public void testPrettyPrinter4() {
assertPrettyPrint(
"function f() {}if (1) {alert();}",
"function f() {\n" +
"}\n" +
"if(1) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint(
"var f = function() {};if (1) {alert();}",
"var f = function() {\n" +
"};\n" +
"if(1) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint(
"(function() {})();if (1) {alert();}",
"(function() {\n" +
"})();\n" +
"if(1) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint(
"(function() {alert();alert();})();if (1) {alert();}",
"(function() {\n" +
" alert();\n" +
" alert()\n" +
"})();\n" +
"if(1) {\n" +
" alert()\n" +
"}\n");
}
public void testTypeAnnotations() {
assertTypeAnnotations(
"/** @constructor */ function Foo(){}",
"/**\n * @return {undefined}\n * @constructor\n */\n"
+ "function Foo() {\n}\n");
}
public void testTypeAnnotationsTypeDef() {
// TODO(johnlenz): It would be nice if there were some way to preserve
// typedefs but currently they are resolved into the basic types in the
// type registry.
assertTypeAnnotations(
"/** @typedef {Array.<number>} */ goog.java.Long;\n"
+ "/** @param {!goog.java.Long} a*/\n"
+ "function f(a){};\n",
"goog.java.Long;\n"
+ "/**\n"
+ " * @param {(Array|null)} a\n"
+ " * @return {undefined}\n"
+ " */\n"
+ "function f(a) {\n}\n");
}
public void testTypeAnnotationsAssign() {
assertTypeAnnotations("/** @constructor */ var Foo = function(){}",
"/**\n * @return {undefined}\n * @constructor\n */\n"
+ "var Foo = function() {\n};\n");
}
public void testTypeAnnotationsNamespace() {
assertTypeAnnotations("var a = {};"
+ "/** @constructor */ a.Foo = function(){}",
"var a = {};\n"
+ "/**\n * @return {undefined}\n * @constructor\n */\n"
+ "a.Foo = function() {\n};\n");
}
public void testTypeAnnotationsMemberSubclass() {
assertTypeAnnotations("var a = {};"
+ "/** @constructor */ a.Foo = function(){};"
+ "/** @constructor \n @extends {a.Foo} */ a.Bar = function(){}",
"var a = {};\n"
+ "/**\n * @return {undefined}\n * @constructor\n */\n"
+ "a.Foo = function() {\n};\n"
+ "/**\n * @return {undefined}\n * @extends {a.Foo}\n"
+ " * @constructor\n */\n"
+ "a.Bar = function() {\n};\n");
}
public void testTypeAnnotationsInterface() {
assertTypeAnnotations("var a = {};"
+ "/** @interface */ a.Foo = function(){};"
+ "/** @interface \n @extends {a.Foo} */ a.Bar = function(){}",
"var a = {};\n"
+ "/**\n * @interface\n */\n"
+ "a.Foo = function() {\n};\n"
+ "/**\n * @extends {a.Foo}\n"
+ " * @interface\n */\n"
+ "a.Bar = function() {\n};\n");
}
public void testTypeAnnotationsMultipleInterface() {
assertTypeAnnotations("var a = {};"
+ "/** @interface */ a.Foo1 = function(){};"
+ "/** @interface */ a.Foo2 = function(){};"
+ "/** @interface \n @extends {a.Foo1} \n @extends {a.Foo2} */"
+ "a.Bar = function(){}",
"var a = {};\n"
+ "/**\n * @interface\n */\n"
+ "a.Foo1 = function() {\n};\n"
+ "/**\n * @interface\n */\n"
+ "a.Foo2 = function() {\n};\n"
+ "/**\n * @extends {a.Foo1}\n"
+ " * @extends {a.Foo2}\n"
+ " * @interface\n */\n"
+ "a.Bar = function() {\n};\n");
}
public void testTypeAnnotationsMember() {
assertTypeAnnotations("var a = {};"
+ "/** @constructor */ a.Foo = function(){}"
+ "/** @param {string} foo\n"
+ " * @return {number} */\n"
+ "a.Foo.prototype.foo = function(foo) { return 3; };"
+ "/** @type {string|undefined} */"
+ "a.Foo.prototype.bar = '';",
"var a = {};\n"
+ "/**\n * @return {undefined}\n * @constructor\n */\n"
+ "a.Foo = function() {\n};\n"
+ "/**\n"
+ " * @param {string} foo\n"
+ " * @return {number}\n"
+ " */\n"
+ "a.Foo.prototype.foo = function(foo) {\n return 3\n};\n"
+ "/** @type {string} */\n"
+ "a.Foo.prototype.bar = \"\";\n");
}
public void testTypeAnnotationsImplements() {
assertTypeAnnotations("var a = {};"
+ "/** @constructor */ a.Foo = function(){};\n"
+ "/** @interface */ a.I = function(){};\n"
+ "/** @interface */ a.I2 = function(){};\n"
+ "/** @constructor \n @extends {a.Foo}\n"
+ " * @implements {a.I} \n @implements {a.I2}\n"
+ "*/ a.Bar = function(){}",
"var a = {};\n"
+ "/**\n * @return {undefined}\n * @constructor\n */\n"
+ "a.Foo = function() {\n};\n"
+ "/**\n * @interface\n */\n"
+ "a.I = function() {\n};\n"
+ "/**\n * @interface\n */\n"
+ "a.I2 = function() {\n};\n"
+ "/**\n * @return {undefined}\n * @extends {a.Foo}\n"
+ " * @implements {a.I}\n"
+ " * @implements {a.I2}\n * @constructor\n */\n"
+ "a.Bar = function() {\n};\n");
}
public void testTypeAnnotationsDispatcher1() {
assertTypeAnnotations(
"var a = {};\n" +
"/** \n" +
" * @constructor \n" +
" * @javadispatch \n" +
" */\n" +
"a.Foo = function(){}",
"var a = {};\n" +
"/**\n" +
" * @return {undefined}\n" +
" * @constructor\n" +
" * @javadispatch\n" +
" */\n" +
"a.Foo = function() {\n" +
"};\n");
}
public void testTypeAnnotationsDispatcher2() {
assertTypeAnnotations(
"var a = {};\n" +
"/** \n" +
" * @constructor \n" +
" */\n" +
"a.Foo = function(){}\n" +
"/**\n" +
" * @javadispatch\n" +
" */\n" +
"a.Foo.prototype.foo = function() {};",
"var a = {};\n" +
"/**\n" +
" * @return {undefined}\n" +
" * @constructor\n" +
" */\n" +
"a.Foo = function() {\n" +
"};\n" +
"/**\n" +
" * @return {undefined}\n" +
" * @javadispatch\n" +
" */\n" +
"a.Foo.prototype.foo = function() {\n" +
"};\n");
}
public void testU2UFunctionTypeAnnotation() {
assertTypeAnnotations(
"/** @type {!Function} */ var x = function() {}",
"/**\n * @constructor\n */\nvar x = function() {\n};\n");
}
public void testEmitUnknownParamTypesAsAllType() {
assertTypeAnnotations(
"var a = function(x) {}",
"/**\n" +
" * @param {*} x\n" +
" * @return {undefined}\n" +
" */\n" +
"var a = function(x) {\n};\n");
}
public void testOptionalTypesAnnotation() {
assertTypeAnnotations(
"/**\n" +
" * @param {string=} x \n" +
" */\n" +
"var a = function(x) {}",
"/**\n" +
" * @param {string=} x\n" +
" * @return {undefined}\n" +
" */\n" +
"var a = function(x) {\n};\n");
}
public void testVariableArgumentsTypesAnnotation() {
assertTypeAnnotations(
"/**\n" +
" * @param {...string} x \n" +
" */\n" +
"var a = function(x) {}",
"/**\n" +
" * @param {...string} x\n" +
" * @return {undefined}\n" +
" */\n" +
"var a = function(x) {\n};\n");
}
public void testTempConstructor() {
assertTypeAnnotations(
"var x = function() {\n/**\n * @constructor\n */\nfunction t1() {}\n" +
" /**\n * @constructor\n */\nfunction t2() {}\n" +
" t1.prototype = t2.prototype}",
"/**\n * @return {undefined}\n */\nvar x = function() {\n" +
" /**\n * @return {undefined}\n * @constructor\n */\n" +
"function t1() {\n }\n" +
" /**\n * @return {undefined}\n * @constructor\n */\n" +
"function t2() {\n }\n" +
" t1.prototype = t2.prototype\n};\n"
);
}
private void assertPrettyPrint(String js, String expected) {
assertEquals(expected,
parsePrint(js, true, false,
CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD));
}
private void assertTypeAnnotations(String js, String expected) {
assertEquals(expected,
parsePrint(js, true, false,
CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD, true));
}
public void testSubtraction() {
Compiler compiler = new Compiler();
Node n = compiler.parseTestCode("x - -4");
assertEquals(0, compiler.getErrorCount());
assertEquals(
"x- -4",
new CodePrinter.Builder(n).setLineLengthThreshold(
CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD).build());
}
public void testFunctionWithCall() {
assertPrint(
"var user = new function() {"
+ "alert(\"foo\")}",
"var user=new function(){"
+ "alert(\"foo\")}");
assertPrint(
"var user = new function() {"
+ "this.name = \"foo\";"
+ "this.local = function(){alert(this.name)};}",
"var user=new function(){"
+ "this.name=\"foo\";"
+ "this.local=function(){alert(this.name)}}");
}
public void testLineLength() {
// list
assertLineLength("var aba,bcb,cdc",
"var aba,bcb," +
"\ncdc");
// operators, and two breaks
assertLineLength(
"\"foo\"+\"bar,baz,bomb\"+\"whee\"+\";long-string\"\n+\"aaa\"",
"\"foo\"+\"bar,baz,bomb\"+" +
"\n\"whee\"+\";long-string\"+" +
"\n\"aaa\"");
// assignment
assertLineLength("var abazaba=1234",
"var abazaba=" +
"\n1234");
// statements
assertLineLength("var abab=1;var bab=2",
"var abab=1;" +
"\nvar bab=2");
// don't break regexes
assertLineLength("var a=/some[reg](ex),with.*we?rd|chars/i;var b=a",
"var a=/some[reg](ex),with.*we?rd|chars/i;" +
"\nvar b=a");
// don't break strings
assertLineLength("var a=\"foo,{bar};baz\";var b=a",
"var a=\"foo,{bar};baz\";" +
"\nvar b=a");
// don't break before post inc/dec
assertLineLength("var a=\"a\";a++;var b=\"bbb\";",
"var a=\"a\";a++;\n" +
"var b=\"bbb\"");
}
private void assertLineLength(String js, String expected) {
assertEquals(expected,
parsePrint(js, false, true, 10));
}
public void testParsePrintParse() {
testReparse("3;");
testReparse("var a = b;");
testReparse("var x, y, z;");
testReparse("try { foo() } catch(e) { bar() }");
testReparse("try { foo() } catch(e) { bar() } finally { stuff() }");
testReparse("try { foo() } finally { stuff() }");
testReparse("throw 'me'");
testReparse("function foo(a) { return a + 4; }");
testReparse("function foo() { return; }");
testReparse("var a = function(a, b) { foo(); return a + b; }");
testReparse("b = [3, 4, 'paul', \"Buchhe it\",,5];");
testReparse("v = (5, 6, 7, 8)");
testReparse("d = 34.0; x = 0; y = .3; z = -22");
testReparse("d = -x; t = !x + ~y;");
testReparse("'hi'; /* just a test */ stuff(a,b) \n" +
" foo(); // and another \n" +
" bar();");
testReparse("a = b++ + ++c; a = b++-++c; a = - --b; a = - ++b;");
testReparse("a++; b= a++; b = ++a; b = a--; b = --a; a+=2; b-=5");
testReparse("a = (2 + 3) * 4;");
testReparse("a = 1 + (2 + 3) + 4;");
testReparse("x = a ? b : c; x = a ? (b,3,5) : (foo(),bar());");
testReparse("a = b | c || d ^ e " +
"&& f & !g != h << i <= j < k >>> l > m * n % !o");
testReparse("a == b; a != b; a === b; a == b == a;" +
" (a == b) == a; a == (b == a);");
testReparse("if (a > b) a = b; if (b < 3) a = 3; else c = 4;");
testReparse("if (a == b) { a++; } if (a == 0) { a++; } else { a --; }");
testReparse("for (var i in a) b += i;");
testReparse("for (var i = 0; i < 10; i++){ b /= 2;" +
" if (b == 2)break;else continue;}");
testReparse("for (x = 0; x < 10; x++) a /= 2;");
testReparse("for (;;) a++;");
testReparse("while(true) { blah(); }while(true) blah();");
testReparse("do stuff(); while(a>b);");
testReparse("[0, null, , true, false, this];");
testReparse("s.replace(/absc/, 'X').replace(/ab/gi, 'Y');");
testReparse("new Foo; new Bar(a, b,c);");
testReparse("with(foo()) { x = z; y = t; } with(bar()) a = z;");
testReparse("delete foo['bar']; delete foo;");
testReparse("var x = { 'a':'paul', 1:'3', 2:(3,4) };");
testReparse("switch(a) { case 2: case 3: stuff(); break;" +
"case 4: morestuff(); break; default: done();}");
testReparse("x = foo['bar'] + foo['my stuff'] + foo[bar] + f.stuff;");
testReparse("a.v = b.v; x['foo'] = y['zoo'];");
testReparse("'test' in x; 3 in x; a in x;");
testReparse("'foo\"bar' + \"foo'c\" + 'stuff\\n and \\\\more'");
testReparse("x.__proto__;");
}
private void testReparse(String code) {
Compiler compiler = new Compiler();
Node parse1 = parse(code);
Node parse2 = parse(new CodePrinter.Builder(parse1).build());
String explanation = parse1.checkTreeEquals(parse2);
assertNull("\nExpected: " + compiler.toSource(parse1) +
"\nResult: " + compiler.toSource(parse2) +
"\n" + explanation, explanation);
}
public void testDoLoopIECompatiblity() {
// Do loops within IFs cause syntax errors in IE6 and IE7.
assertPrint("function f(){if(e1){do foo();while(e2)}else foo()}",
"function f(){if(e1){do foo();while(e2)}else foo()}");
assertPrint("function f(){if(e1)do foo();while(e2)else foo()}",
"function f(){if(e1){do foo();while(e2)}else foo()}");
assertPrint("if(x){do{foo()}while(y)}else bar()",
"if(x){do foo();while(y)}else bar()");
assertPrint("if(x)do{foo()}while(y);else bar()",
"if(x){do foo();while(y)}else bar()");
assertPrint("if(x){do{foo()}while(y)}",
"if(x){do foo();while(y)}");
assertPrint("if(x)do{foo()}while(y);",
"if(x){do foo();while(y)}");
assertPrint("if(x)A:do{foo()}while(y);",
"if(x){A:do foo();while(y)}");
assertPrint("var i = 0;a: do{b: do{i++;break b;} while(0);} while(0);",
"var i=0;a:do{b:do{i++;break b}while(0)}while(0)");
}
public void testFunctionSafariCompatiblity() {
// Functions within IFs cause syntax errors on Safari.
assertPrint("function f(){if(e1){function goo(){return true}}else foo()}",
"function f(){if(e1){function goo(){return true}}else foo()}");
assertPrint("function f(){if(e1)function goo(){return true}else foo()}",
"function f(){if(e1){function goo(){return true}}else foo()}");
assertPrint("if(e1){function goo(){return true}}",
"if(e1){function goo(){return true}}");
assertPrint("if(e1)function goo(){return true}",
"if(e1){function goo(){return true}}");
assertPrint("if(e1)A:function goo(){return true}",
"if(e1){A:function goo(){return true}}");
}
public void testExponents() {
assertPrintNumber("1", 1);
assertPrintNumber("10", 10);
assertPrintNumber("100", 100);
assertPrintNumber("1E3", 1000);
assertPrintNumber("1E4", 10000);
assertPrintNumber("1E5", 100000);
assertPrintNumber("-1", -1);
assertPrintNumber("-10", -10);
assertPrintNumber("-100", -100);
assertPrintNumber("-1E3", -1000);
assertPrintNumber("-12341234E4", -123412340000L);
assertPrintNumber("1E18", 1000000000000000000L);
assertPrintNumber("1E5", 100000.0);
assertPrintNumber("100000.1", 100000.1);
assertPrintNumber("1.0E-6", 0.000001);
}
// Make sure to test as both a String and a Node, because
// negative numbers do not parse consistently from strings.
private void assertPrintNumber(String expected, double number) {
assertPrint(String.valueOf(number), expected);
assertPrintNode(expected, Node.newNumber(number));
}
private void assertPrintNumber(String expected, int number) {
assertPrint(String.valueOf(number), expected);
assertPrintNode(expected, Node.newNumber(number));
}
public void testDirectEval() {
assertPrint("eval('1');", "eval(\"1\")");
}
public void testIndirectEval() {
Node n = parse("eval('1');");
assertPrintNode("eval(\"1\")", n);
n.getFirstChild().getFirstChild().getFirstChild().putBooleanProp(
Node.DIRECT_EVAL, false);
assertPrintNode("(0,eval)(\"1\")", n);
}
public void testFreeCall1() {
assertPrint("foo(a);", "foo(a)");
assertPrint("x.foo(a);", "x.foo(a)");
}
public void testFreeCall2() {
Node n = parse("foo(a);");
assertPrintNode("foo(a)", n);
Node call = n.getFirstChild().getFirstChild();
assertTrue(call.getType() == Token.CALL);
call.putBooleanProp(Node.FREE_CALL, true);
assertPrintNode("foo(a)", n);
}
public void testFreeCall3() {
Node n = parse("x.foo(a);");
assertPrintNode("x.foo(a)", n);
Node call = n.getFirstChild().getFirstChild();
assertTrue(call.getType() == Token.CALL);
call.putBooleanProp(Node.FREE_CALL, true);
assertPrintNode("(0,x.foo)(a)", n);
}
public void testPrintScript() {
// Verify that SCRIPT nodes not marked as synthetic are printed as
// blocks.
Node ast = new Node(Token.SCRIPT,
new Node(Token.EXPR_RESULT, Node.newString("f")),
new Node(Token.EXPR_RESULT, Node.newString("g")));
String result = new CodePrinter.Builder(ast).setPrettyPrint(true).build();
assertEquals("\"f\";\n\"g\";\n", result);
}
public void testObjectLit() {
assertPrint("({x:1})", "({x:1})");
assertPrint("var x=({x:1})", "var x={x:1}");
assertPrint("var x={'x':1}", "var x={\"x\":1}");
assertPrint("var x={1:1}", "var x={1:1}");
}
public void testObjectLit2() {
assertPrint("var x={1:1}", "var x={1:1}");
assertPrint("var x={'1':1}", "var x={1:1}");
assertPrint("var x={'1.0':1}", "var x={\"1.0\":1}");
assertPrint("var x={1.5:1}", "var x={\"1.5\":1}");
}
public void testObjectLit3() {
assertPrint("var x={3E9:1}",
"var x={3E9:1}");
assertPrint("var x={'3000000000':1}", // More than 31 bits
"var x={3E9:1}");
assertPrint("var x={'3000000001':1}",
"var x={3000000001:1}");
assertPrint("var x={'6000000001':1}", // More than 32 bits
"var x={6000000001:1}");
assertPrint("var x={\"12345678901234567\":1}", // More than 53 bits
"var x={\"12345678901234567\":1}");
}
public void testObjectLit4() {
// More than 128 bits.
assertPrint(
"var x={\"123456789012345671234567890123456712345678901234567\":1}",
"var x={\"123456789012345671234567890123456712345678901234567\":1}");
}
public void testGetter() {
assertPrint("var x = {}", "var x={}");
assertPrint("var x = {get a() {return 1}}", "var x={get a(){return 1}}");
assertPrint(
"var x = {get a() {}, get b(){}}",
"var x={get a(){},get b(){}}");
assertPrint(
"var x = {get 'a'() {return 1}}",
"var x={get \"a\"(){return 1}}");
assertPrint(
"var x = {get 1() {return 1}}",
"var x={get 1(){return 1}}");
assertPrint(
"var x = {get \"()\"() {return 1}}",
"var x={get \"()\"(){return 1}}");
}
public void testSetter() {
assertPrint("var x = {}", "var x={}");
assertPrint(
"var x = {set a(y) {return 1}}",
"var x={set a(y){return 1}}");
assertPrint(
"var x = {get 'a'() {return 1}}",
"var x={get \"a\"(){return 1}}");
assertPrint(
"var x = {set 1(y) {return 1}}",
"var x={set 1(y){return 1}}");
assertPrint(
"var x = {set \"(x)\"(y) {return 1}}",
"var x={set \"(x)\"(y){return 1}}");
}
public void testNegCollapse() {
// Collapse the negative symbol on numbers at generation time,
// to match the Rhino behavior.
assertPrint("var x = - - 2;", "var x=2");
assertPrint("var x = - (2);", "var x=-2");
}
public void testStrict() {
String result = parsePrint("var x", false, false, 0, false, true);
assertEquals("'use strict';var x", result);
}
public void testArrayLiteral() {
assertPrint("var x = [,];","var x=[,]");
assertPrint("var x = [,,];","var x=[,,]");
assertPrint("var x = [,s,,];","var x=[,s,,]");
assertPrint("var x = [,s];","var x=[,s]");
assertPrint("var x = [s,];","var x=[s]");
}
public void testZero() {
assertPrint("var x ='\\0';", "var x=\"\\000\"");
assertPrint("var x ='\\x00';", "var x=\"\\000\"");
assertPrint("var x ='\\u0000';", "var x=\"\\000\"");
assertPrint("var x ='\\u00003';", "var x=\"\\0003\"");
}
public void testUnicode() {
assertPrint("var x ='\\x0f';", "var x=\"\\u000f\"");
assertPrint("var x ='\\x68';", "var x=\"h\"");
assertPrint("var x ='\\x7f';", "var x=\"\\u007f\"");
}
} | // You are a professional Java test case writer, please create a test case named `testZero` for the issue `Closure-486`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-486
//
// ## Issue-Title:
// String escaping mishandles null byte
//
// ## Issue-Description:
// **What steps will reproduce the problem?**
// 1. Run:
// var x = "\u00003"; if (x.length < 2) { alert("fail"); } else { alert("win"); }
// 2. Compile and run
//
// **What is the expected output? What do you see instead?**
// "win" is expected. "fail" is observed
//
// **What version of the product are you using? On what operating system?**
// r1167 on OS x 10.6
//
// **Please provide any additional information below.**
// The problem is here: http://code.google.com/p/closure-compiler/source/browse/trunk/src/com/google/javascript/jscomp/CodeGenerator.java#1015
//
// Here's a patch that fixes it:
// $ svn diff
// Index: src/com/google/javascript/jscomp/CodeGenerator.java
// ===================================================================
// --- src/com/google/javascript/jscomp/CodeGenerator.java (revision 1167)
// +++ src/com/google/javascript/jscomp/CodeGenerator.java (working copy)
// @@ -1012,7 +1012,7 @@
// for (int i = 0; i < s.length(); i++) {
// char c = s.charAt(i);
// switch (c) {
// - case '\0': sb.append("\\0"); break;
// + case '\0': sb.append("\\000"); break;
// case '\n': sb.append("\\n"); break;
// case '\r': sb.append("\\r"); break;
// case '\t': sb.append("\\t"); break;
//
// You could also lookahead and output "\\000" only if the following char is 0-7 (octal valid) and otherwise output "\\0". Is 2 bytes worth the complexity?
//
//
public void testZero() {
| 1,234 | 65 | 1,229 | test/com/google/javascript/jscomp/CodePrinterTest.java | test | ```markdown
## Issue-ID: Closure-486
## Issue-Title:
String escaping mishandles null byte
## Issue-Description:
**What steps will reproduce the problem?**
1. Run:
var x = "\u00003"; if (x.length < 2) { alert("fail"); } else { alert("win"); }
2. Compile and run
**What is the expected output? What do you see instead?**
"win" is expected. "fail" is observed
**What version of the product are you using? On what operating system?**
r1167 on OS x 10.6
**Please provide any additional information below.**
The problem is here: http://code.google.com/p/closure-compiler/source/browse/trunk/src/com/google/javascript/jscomp/CodeGenerator.java#1015
Here's a patch that fixes it:
$ svn diff
Index: src/com/google/javascript/jscomp/CodeGenerator.java
===================================================================
--- src/com/google/javascript/jscomp/CodeGenerator.java (revision 1167)
+++ src/com/google/javascript/jscomp/CodeGenerator.java (working copy)
@@ -1012,7 +1012,7 @@
for (int i = 0; i < s.length(); i++) {
char c = s.charAt(i);
switch (c) {
- case '\0': sb.append("\\0"); break;
+ case '\0': sb.append("\\000"); break;
case '\n': sb.append("\\n"); break;
case '\r': sb.append("\\r"); break;
case '\t': sb.append("\\t"); break;
You could also lookahead and output "\\000" only if the following char is 0-7 (octal valid) and otherwise output "\\0". Is 2 bytes worth the complexity?
```
You are a professional Java test case writer, please create a test case named `testZero` for the issue `Closure-486`, utilizing the provided issue report information and the following function signature.
```java
public void testZero() {
```
| 1,229 | [
"com.google.javascript.jscomp.CodeGenerator"
] | a0a8a95cf1149923ba0f7ff8c9bcb53a389fec891e88071d3aac134866b4f39c | public void testZero() | // You are a professional Java test case writer, please create a test case named `testZero` for the issue `Closure-486`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-486
//
// ## Issue-Title:
// String escaping mishandles null byte
//
// ## Issue-Description:
// **What steps will reproduce the problem?**
// 1. Run:
// var x = "\u00003"; if (x.length < 2) { alert("fail"); } else { alert("win"); }
// 2. Compile and run
//
// **What is the expected output? What do you see instead?**
// "win" is expected. "fail" is observed
//
// **What version of the product are you using? On what operating system?**
// r1167 on OS x 10.6
//
// **Please provide any additional information below.**
// The problem is here: http://code.google.com/p/closure-compiler/source/browse/trunk/src/com/google/javascript/jscomp/CodeGenerator.java#1015
//
// Here's a patch that fixes it:
// $ svn diff
// Index: src/com/google/javascript/jscomp/CodeGenerator.java
// ===================================================================
// --- src/com/google/javascript/jscomp/CodeGenerator.java (revision 1167)
// +++ src/com/google/javascript/jscomp/CodeGenerator.java (working copy)
// @@ -1012,7 +1012,7 @@
// for (int i = 0; i < s.length(); i++) {
// char c = s.charAt(i);
// switch (c) {
// - case '\0': sb.append("\\0"); break;
// + case '\0': sb.append("\\000"); break;
// case '\n': sb.append("\\n"); break;
// case '\r': sb.append("\\r"); break;
// case '\t': sb.append("\\t"); break;
//
// You could also lookahead and output "\\000" only if the following char is 0-7 (octal valid) and otherwise output "\\0". Is 2 bytes worth the complexity?
//
//
| Closure | /*
* Copyright 2004 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import com.google.javascript.jscomp.CompilerOptions.LanguageMode;
import com.google.javascript.rhino.Node;
import com.google.javascript.rhino.Token;
import junit.framework.TestCase;
public class CodePrinterTest extends TestCase {
static Node parse(String js) {
return parse(js, false);
}
static Node parse(String js, boolean checkTypes) {
Compiler compiler = new Compiler();
CompilerOptions options = new CompilerOptions();
// Allow getters and setters.
options.setLanguageIn(LanguageMode.ECMASCRIPT5);
compiler.initOptions(options);
Node n = compiler.parseTestCode(js);
if (checkTypes) {
DefaultPassConfig passConfig = new DefaultPassConfig(null);
CompilerPass typeResolver = passConfig.resolveTypes.create(compiler);
Node externs = new Node(Token.SCRIPT);
externs.setIsSyntheticBlock(true);
Node externAndJsRoot = new Node(Token.BLOCK, externs, n);
externAndJsRoot.setIsSyntheticBlock(true);
typeResolver.process(externs, n);
CompilerPass inferTypes = passConfig.inferTypes.create(compiler);
inferTypes.process(externs, n);
}
checkUnexpectedErrorsOrWarnings(compiler, 0);
return n;
}
private static void checkUnexpectedErrorsOrWarnings(
Compiler compiler, int expected) {
int actual = compiler.getErrors().length + compiler.getWarnings().length;
if (actual != expected) {
String msg = "";
for (JSError err : compiler.getErrors()) {
msg += "Error:" + err.toString() + "\n";
}
for (JSError err : compiler.getWarnings()) {
msg += "Warning:" + err.toString() + "\n";
}
assertEquals("Unexpected warnings or errors.\n " + msg, expected, actual);
}
}
String parsePrint(String js, boolean prettyprint, int lineThreshold) {
return new CodePrinter.Builder(parse(js)).setPrettyPrint(prettyprint)
.setLineLengthThreshold(lineThreshold).build();
}
String parsePrint(String js, boolean prettyprint, boolean lineBreak,
int lineThreshold) {
return new CodePrinter.Builder(parse(js)).setPrettyPrint(prettyprint)
.setLineLengthThreshold(lineThreshold).setLineBreak(lineBreak).build();
}
String parsePrint(String js, boolean prettyprint, boolean lineBreak,
int lineThreshold, boolean outputTypes) {
return new CodePrinter.Builder(parse(js, true)).setPrettyPrint(prettyprint)
.setOutputTypes(outputTypes)
.setLineLengthThreshold(lineThreshold).setLineBreak(lineBreak)
.build();
}
String parsePrint(String js, boolean prettyprint, boolean lineBreak,
int lineThreshold, boolean outputTypes,
boolean tagAsStrict) {
return new CodePrinter.Builder(parse(js, true)).setPrettyPrint(prettyprint)
.setOutputTypes(outputTypes)
.setLineLengthThreshold(lineThreshold).setLineBreak(lineBreak)
.setTagAsStrict(tagAsStrict)
.build();
}
String printNode(Node n) {
return new CodePrinter.Builder(n).setLineLengthThreshold(
CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD).build();
}
void assertPrintNode(String expectedJs, Node ast) {
assertEquals(expectedJs, printNode(ast));
}
public void testPrint() {
assertPrint("10 + a + b", "10+a+b");
assertPrint("10 + (30*50)", "10+30*50");
assertPrint("with(x) { x + 3; }", "with(x)x+3");
assertPrint("\"aa'a\"", "\"aa'a\"");
assertPrint("\"aa\\\"a\"", "'aa\"a'");
assertPrint("function foo()\n{return 10;}", "function foo(){return 10}");
assertPrint("a instanceof b", "a instanceof b");
assertPrint("typeof(a)", "typeof a");
assertPrint(
"var foo = x ? { a : 1 } : {a: 3, b:4, \"default\": 5, \"foo-bar\": 6}",
"var foo=x?{a:1}:{a:3,b:4,\"default\":5,\"foo-bar\":6}");
// Safari: needs ';' at the end of a throw statement
assertPrint("function foo(){throw 'error';}",
"function foo(){throw\"error\";}");
// Safari 3 needs a "{" around a single function
assertPrint("if (true) function foo(){return}",
"if(true){function foo(){return}}");
assertPrint("var x = 10; { var y = 20; }", "var x=10;var y=20");
assertPrint("while (x-- > 0);", "while(x-- >0);");
assertPrint("x-- >> 1", "x-- >>1");
assertPrint("(function () {})(); ",
"(function(){})()");
// Associativity
assertPrint("var a,b,c,d;a || (b&& c) && (a || d)",
"var a,b,c,d;a||b&&c&&(a||d)");
assertPrint("var a,b,c; a || (b || c); a * (b * c); a | (b | c)",
"var a,b,c;a||b||c;a*b*c;a|b|c");
assertPrint("var a,b,c; a / b / c;a / (b / c); a - (b - c);",
"var a,b,c;a/b/c;a/(b/c);a-(b-c)");
assertPrint("var a,b; a = b = 3;",
"var a,b;a=b=3");
assertPrint("var a,b,c,d; a = (b = c = (d = 3));",
"var a,b,c,d;a=b=c=d=3");
assertPrint("var a,b,c; a += (b = c += 3);",
"var a,b,c;a+=b=c+=3");
assertPrint("var a,b,c; a *= (b -= c);",
"var a,b,c;a*=b-=c");
// Break scripts
assertPrint("'<script>'", "\"<script>\"");
assertPrint("'</script>'", "\"<\\/script>\"");
assertPrint("\"</script> </SCRIPT>\"", "\"<\\/script> <\\/SCRIPT>\"");
assertPrint("'-->'", "\"--\\>\"");
assertPrint("']]>'", "\"]]\\>\"");
assertPrint("' --></script>'", "\" --\\><\\/script>\"");
assertPrint("/--> <\\/script>/g", "/--\\> <\\/script>/g");
// Break HTML start comments. Certain versions of Webkit
// begin an HTML comment when they see this.
assertPrint("'<!-- I am a string -->'", "\"<\\!-- I am a string --\\>\"");
// Precedence
assertPrint("a ? delete b[0] : 3", "a?delete b[0]:3");
assertPrint("(delete a[0])/10", "delete a[0]/10");
// optional '()' for new
// simple new
assertPrint("new A", "new A");
assertPrint("new A()", "new A");
assertPrint("new A('x')", "new A(\"x\")");
// calling instance method directly after new
assertPrint("new A().a()", "(new A).a()");
assertPrint("(new A).a()", "(new A).a()");
// this case should be fixed
assertPrint("new A('y').a()", "(new A(\"y\")).a()");
// internal class
assertPrint("new A.B", "new A.B");
assertPrint("new A.B()", "new A.B");
assertPrint("new A.B('z')", "new A.B(\"z\")");
// calling instance method directly after new internal class
assertPrint("(new A.B).a()", "(new A.B).a()");
assertPrint("new A.B().a()", "(new A.B).a()");
// this case should be fixed
assertPrint("new A.B('w').a()", "(new A.B(\"w\")).a()");
// Operators: make sure we don't convert binary + and unary + into ++
assertPrint("x + +y", "x+ +y");
assertPrint("x - (-y)", "x- -y");
assertPrint("x++ +y", "x++ +y");
assertPrint("x-- -y", "x-- -y");
assertPrint("x++ -y", "x++-y");
// Label
assertPrint("foo:for(;;){break foo;}", "foo:for(;;)break foo");
assertPrint("foo:while(1){continue foo;}", "foo:while(1)continue foo");
// Object literals.
assertPrint("({})", "({})");
assertPrint("var x = {};", "var x={}");
assertPrint("({}).x", "({}).x");
assertPrint("({})['x']", "({})[\"x\"]");
assertPrint("({}) instanceof Object", "({})instanceof Object");
assertPrint("({}) || 1", "({})||1");
assertPrint("1 || ({})", "1||{}");
assertPrint("({}) ? 1 : 2", "({})?1:2");
assertPrint("0 ? ({}) : 2", "0?{}:2");
assertPrint("0 ? 1 : ({})", "0?1:{}");
assertPrint("typeof ({})", "typeof{}");
assertPrint("f({})", "f({})");
// Anonymous function expressions.
assertPrint("(function(){})", "(function(){})");
assertPrint("(function(){})()", "(function(){})()");
assertPrint("(function(){})instanceof Object",
"(function(){})instanceof Object");
assertPrint("(function(){}).bind().call()",
"(function(){}).bind().call()");
assertPrint("var x = function() { };", "var x=function(){}");
assertPrint("var x = function() { }();", "var x=function(){}()");
assertPrint("(function() {}), 2", "(function(){}),2");
// Name functions expression.
assertPrint("(function f(){})", "(function f(){})");
// Function declaration.
assertPrint("function f(){}", "function f(){}");
// Make sure we don't treat non-latin character escapes as raw strings.
assertPrint("({ 'a': 4, '\\u0100': 4 })", "({\"a\":4,\"\\u0100\":4})");
assertPrint("({ a: 4, '\\u0100': 4 })", "({a:4,\"\\u0100\":4})");
// Test if statement and for statements with single statements in body.
assertPrint("if (true) { alert();}", "if(true)alert()");
assertPrint("if (false) {} else {alert(\"a\");}",
"if(false);else alert(\"a\")");
assertPrint("for(;;) { alert();};", "for(;;)alert()");
assertPrint("do { alert(); } while(true);",
"do alert();while(true)");
assertPrint("myLabel: { alert();}",
"myLabel:alert()");
assertPrint("myLabel: for(;;) continue myLabel;",
"myLabel:for(;;)continue myLabel");
// Test nested var statement
assertPrint("if (true) var x; x = 4;", "if(true)var x;x=4");
// Non-latin identifier. Make sure we keep them escaped.
assertPrint("\\u00fb", "\\u00fb");
assertPrint("\\u00fa=1", "\\u00fa=1");
assertPrint("function \\u00f9(){}", "function \\u00f9(){}");
assertPrint("x.\\u00f8", "x.\\u00f8");
assertPrint("x.\\u00f8", "x.\\u00f8");
assertPrint("abc\\u4e00\\u4e01jkl", "abc\\u4e00\\u4e01jkl");
// Test the right-associative unary operators for spurious parens
assertPrint("! ! true", "!!true");
assertPrint("!(!(true))", "!!true");
assertPrint("typeof(void(0))", "typeof void 0");
assertPrint("typeof(void(!0))", "typeof void!0");
assertPrint("+ - + + - + 3", "+-+ +-+3"); // chained unary plus/minus
assertPrint("+(--x)", "+--x");
assertPrint("-(++x)", "-++x");
// needs a space to prevent an ambiguous parse
assertPrint("-(--x)", "- --x");
assertPrint("!(~~5)", "!~~5");
assertPrint("~(a/b)", "~(a/b)");
// Preserve parens to overcome greedy binding of NEW
assertPrint("new (foo.bar()).factory(baz)", "new (foo.bar().factory)(baz)");
assertPrint("new (bar()).factory(baz)", "new (bar().factory)(baz)");
assertPrint("new (new foobar(x)).factory(baz)",
"new (new foobar(x)).factory(baz)");
// Make sure that HOOK is right associative
assertPrint("a ? b : (c ? d : e)", "a?b:c?d:e");
assertPrint("a ? (b ? c : d) : e", "a?b?c:d:e");
assertPrint("(a ? b : c) ? d : e", "(a?b:c)?d:e");
// Test nested ifs
assertPrint("if (x) if (y); else;", "if(x)if(y);else;");
// Test comma.
assertPrint("a,b,c", "a,b,c");
assertPrint("(a,b),c", "a,b,c");
assertPrint("a,(b,c)", "a,b,c");
assertPrint("x=a,b,c", "x=a,b,c");
assertPrint("x=(a,b),c", "x=(a,b),c");
assertPrint("x=a,(b,c)", "x=a,b,c");
assertPrint("x=a,y=b,z=c", "x=a,y=b,z=c");
assertPrint("x=(a,y=b,z=c)", "x=(a,y=b,z=c)");
assertPrint("x=[a,b,c,d]", "x=[a,b,c,d]");
assertPrint("x=[(a,b,c),d]", "x=[(a,b,c),d]");
assertPrint("x=[(a,(b,c)),d]", "x=[(a,b,c),d]");
assertPrint("x=[a,(b,c,d)]", "x=[a,(b,c,d)]");
assertPrint("var x=(a,b)", "var x=(a,b)");
assertPrint("var x=a,b,c", "var x=a,b,c");
assertPrint("var x=(a,b),c", "var x=(a,b),c");
assertPrint("var x=a,b=(c,d)", "var x=a,b=(c,d)");
assertPrint("foo(a,b,c,d)", "foo(a,b,c,d)");
assertPrint("foo((a,b,c),d)", "foo((a,b,c),d)");
assertPrint("foo((a,(b,c)),d)", "foo((a,b,c),d)");
assertPrint("f(a+b,(c,d,(e,f,g)))", "f(a+b,(c,d,e,f,g))");
assertPrint("({}) , 1 , 2", "({}),1,2");
assertPrint("({}) , {} , {}", "({}),{},{}");
// EMPTY nodes
assertPrint("if (x){}", "if(x);");
assertPrint("if(x);", "if(x);");
assertPrint("if(x)if(y);", "if(x)if(y);");
assertPrint("if(x){if(y);}", "if(x)if(y);");
assertPrint("if(x){if(y){};;;}", "if(x)if(y);");
assertPrint("if(x){;;function y(){};;}", "if(x){function y(){}}");
}
public void testPrintArray() {
assertPrint("[void 0, void 0]", "[void 0,void 0]");
assertPrint("[undefined, undefined]", "[undefined,undefined]");
assertPrint("[ , , , undefined]", "[,,,undefined]");
assertPrint("[ , , , 0]", "[,,,0]");
}
public void testHook() {
assertPrint("a ? b = 1 : c = 2", "a?b=1:c=2");
assertPrint("x = a ? b = 1 : c = 2", "x=a?b=1:c=2");
assertPrint("(x = a) ? b = 1 : c = 2", "(x=a)?b=1:c=2");
assertPrint("x, a ? b = 1 : c = 2", "x,a?b=1:c=2");
assertPrint("x, (a ? b = 1 : c = 2)", "x,a?b=1:c=2");
assertPrint("(x, a) ? b = 1 : c = 2", "(x,a)?b=1:c=2");
assertPrint("a ? (x, b) : c = 2", "a?(x,b):c=2");
assertPrint("a ? b = 1 : (x,c)", "a?b=1:(x,c)");
assertPrint("a ? b = 1 : c = 2 + x", "a?b=1:c=2+x");
assertPrint("(a ? b = 1 : c = 2) + x", "(a?b=1:c=2)+x");
assertPrint("a ? b = 1 : (c = 2) + x", "a?b=1:(c=2)+x");
assertPrint("a ? (b?1:2) : 3", "a?b?1:2:3");
}
public void testPrintInOperatorInForLoop() {
// Check for in expression in for's init expression.
// Check alone, with + (higher precedence), with ?: (lower precedence),
// and with conditional.
assertPrint("var a={}; for (var i = (\"length\" in a); i;) {}",
"var a={};for(var i=(\"length\"in a);i;);");
assertPrint("var a={}; for (var i = (\"length\" in a) ? 0 : 1; i;) {}",
"var a={};for(var i=(\"length\"in a)?0:1;i;);");
assertPrint("var a={}; for (var i = (\"length\" in a) + 1; i;) {}",
"var a={};for(var i=(\"length\"in a)+1;i;);");
assertPrint("var a={};for (var i = (\"length\" in a|| \"size\" in a);;);",
"var a={};for(var i=(\"length\"in a)||(\"size\"in a);;);");
assertPrint("var a={};for (var i = a || a || (\"size\" in a);;);",
"var a={};for(var i=a||a||(\"size\"in a);;);");
// Test works with unary operators and calls.
assertPrint("var a={}; for (var i = -(\"length\" in a); i;) {}",
"var a={};for(var i=-(\"length\"in a);i;);");
assertPrint("var a={};function b_(p){ return p;};" +
"for(var i=1,j=b_(\"length\" in a);;) {}",
"var a={};function b_(p){return p}" +
"for(var i=1,j=b_(\"length\"in a);;);");
// Test we correctly handle an in operator in the test clause.
assertPrint("var a={}; for (;(\"length\" in a);) {}",
"var a={};for(;\"length\"in a;);");
}
public void testLiteralProperty() {
assertPrint("(64).toString()", "(64).toString()");
}
private void assertPrint(String js, String expected) {
parse(expected); // validate the expected string is valid js
assertEquals(expected,
parsePrint(js, false, CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD));
}
// Make sure that the code generator doesn't associate an
// else clause with the wrong if clause.
public void testAmbiguousElseClauses() {
assertPrintNode("if(x)if(y);else;",
new Node(Token.IF,
Node.newString(Token.NAME, "x"),
new Node(Token.BLOCK,
new Node(Token.IF,
Node.newString(Token.NAME, "y"),
new Node(Token.BLOCK),
// ELSE clause for the inner if
new Node(Token.BLOCK)))));
assertPrintNode("if(x){if(y);}else;",
new Node(Token.IF,
Node.newString(Token.NAME, "x"),
new Node(Token.BLOCK,
new Node(Token.IF,
Node.newString(Token.NAME, "y"),
new Node(Token.BLOCK))),
// ELSE clause for the outer if
new Node(Token.BLOCK)));
assertPrintNode("if(x)if(y);else{if(z);}else;",
new Node(Token.IF,
Node.newString(Token.NAME, "x"),
new Node(Token.BLOCK,
new Node(Token.IF,
Node.newString(Token.NAME, "y"),
new Node(Token.BLOCK),
new Node(Token.BLOCK,
new Node(Token.IF,
Node.newString(Token.NAME, "z"),
new Node(Token.BLOCK))))),
// ELSE clause for the outermost if
new Node(Token.BLOCK)));
}
public void testLineBreak() {
// line break after function if in a statement context
assertLineBreak("function a() {}\n" +
"function b() {}",
"function a(){}\n" +
"function b(){}\n");
// line break after ; after a function
assertLineBreak("var a = {};\n" +
"a.foo = function () {}\n" +
"function b() {}",
"var a={};a.foo=function(){};\n" +
"function b(){}\n");
// break after comma after a function
assertLineBreak("var a = {\n" +
" b: function() {},\n" +
" c: function() {}\n" +
"};\n" +
"alert(a);",
"var a={b:function(){},\n" +
"c:function(){}};\n" +
"alert(a)");
}
private void assertLineBreak(String js, String expected) {
assertEquals(expected,
parsePrint(js, false, true,
CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD));
}
public void testPrettyPrinter() {
// Ensure that the pretty printer inserts line breaks at appropriate
// places.
assertPrettyPrint("(function(){})();","(function() {\n})();\n");
assertPrettyPrint("var a = (function() {});alert(a);",
"var a = function() {\n};\nalert(a);\n");
// Check we correctly handle putting brackets around all if clauses so
// we can put breakpoints inside statements.
assertPrettyPrint("if (1) {}",
"if(1) {\n" +
"}\n");
assertPrettyPrint("if (1) {alert(\"\");}",
"if(1) {\n" +
" alert(\"\")\n" +
"}\n");
assertPrettyPrint("if (1)alert(\"\");",
"if(1) {\n" +
" alert(\"\")\n" +
"}\n");
assertPrettyPrint("if (1) {alert();alert();}",
"if(1) {\n" +
" alert();\n" +
" alert()\n" +
"}\n");
// Don't add blocks if they weren't there already.
assertPrettyPrint("label: alert();",
"label:alert();\n");
// But if statements and loops get blocks automagically.
assertPrettyPrint("if (1) alert();",
"if(1) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint("for (;;) alert();",
"for(;;) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint("while (1) alert();",
"while(1) {\n" +
" alert()\n" +
"}\n");
// Do we put else clauses in blocks?
assertPrettyPrint("if (1) {} else {alert(a);}",
"if(1) {\n" +
"}else {\n alert(a)\n}\n");
// Do we add blocks to else clauses?
assertPrettyPrint("if (1) alert(a); else alert(b);",
"if(1) {\n" +
" alert(a)\n" +
"}else {\n" +
" alert(b)\n" +
"}\n");
// Do we put for bodies in blocks?
assertPrettyPrint("for(;;) { alert();}",
"for(;;) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint("for(;;) {}",
"for(;;) {\n" +
"}\n");
assertPrettyPrint("for(;;) { alert(); alert(); }",
"for(;;) {\n" +
" alert();\n" +
" alert()\n" +
"}\n");
// How about do loops?
assertPrettyPrint("do { alert(); } while(true);",
"do {\n" +
" alert()\n" +
"}while(true);\n");
// label?
assertPrettyPrint("myLabel: { alert();}",
"myLabel: {\n" +
" alert()\n" +
"}\n");
// Don't move the label on a loop, because then break {label} and
// continue {label} won't work.
assertPrettyPrint("myLabel: for(;;) continue myLabel;",
"myLabel:for(;;) {\n" +
" continue myLabel\n" +
"}\n");
assertPrettyPrint("var a;", "var a;\n");
}
public void testPrettyPrinter2() {
assertPrettyPrint(
"if(true) f();",
"if(true) {\n" +
" f()\n" +
"}\n");
assertPrettyPrint(
"if (true) { f() } else { g() }",
"if(true) {\n" +
" f()\n" +
"}else {\n" +
" g()\n" +
"}\n");
assertPrettyPrint(
"if(true) f(); for(;;) g();",
"if(true) {\n" +
" f()\n" +
"}\n" +
"for(;;) {\n" +
" g()\n" +
"}\n");
}
public void testPrettyPrinter3() {
assertPrettyPrint(
"try {} catch(e) {}if (1) {alert();alert();}",
"try {\n" +
"}catch(e) {\n" +
"}\n" +
"if(1) {\n" +
" alert();\n" +
" alert()\n" +
"}\n");
assertPrettyPrint(
"try {} finally {}if (1) {alert();alert();}",
"try {\n" +
"}finally {\n" +
"}\n" +
"if(1) {\n" +
" alert();\n" +
" alert()\n" +
"}\n");
assertPrettyPrint(
"try {} catch(e) {} finally {} if (1) {alert();alert();}",
"try {\n" +
"}catch(e) {\n" +
"}finally {\n" +
"}\n" +
"if(1) {\n" +
" alert();\n" +
" alert()\n" +
"}\n");
}
public void testPrettyPrinter4() {
assertPrettyPrint(
"function f() {}if (1) {alert();}",
"function f() {\n" +
"}\n" +
"if(1) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint(
"var f = function() {};if (1) {alert();}",
"var f = function() {\n" +
"};\n" +
"if(1) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint(
"(function() {})();if (1) {alert();}",
"(function() {\n" +
"})();\n" +
"if(1) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint(
"(function() {alert();alert();})();if (1) {alert();}",
"(function() {\n" +
" alert();\n" +
" alert()\n" +
"})();\n" +
"if(1) {\n" +
" alert()\n" +
"}\n");
}
public void testTypeAnnotations() {
assertTypeAnnotations(
"/** @constructor */ function Foo(){}",
"/**\n * @return {undefined}\n * @constructor\n */\n"
+ "function Foo() {\n}\n");
}
public void testTypeAnnotationsTypeDef() {
// TODO(johnlenz): It would be nice if there were some way to preserve
// typedefs but currently they are resolved into the basic types in the
// type registry.
assertTypeAnnotations(
"/** @typedef {Array.<number>} */ goog.java.Long;\n"
+ "/** @param {!goog.java.Long} a*/\n"
+ "function f(a){};\n",
"goog.java.Long;\n"
+ "/**\n"
+ " * @param {(Array|null)} a\n"
+ " * @return {undefined}\n"
+ " */\n"
+ "function f(a) {\n}\n");
}
public void testTypeAnnotationsAssign() {
assertTypeAnnotations("/** @constructor */ var Foo = function(){}",
"/**\n * @return {undefined}\n * @constructor\n */\n"
+ "var Foo = function() {\n};\n");
}
public void testTypeAnnotationsNamespace() {
assertTypeAnnotations("var a = {};"
+ "/** @constructor */ a.Foo = function(){}",
"var a = {};\n"
+ "/**\n * @return {undefined}\n * @constructor\n */\n"
+ "a.Foo = function() {\n};\n");
}
public void testTypeAnnotationsMemberSubclass() {
assertTypeAnnotations("var a = {};"
+ "/** @constructor */ a.Foo = function(){};"
+ "/** @constructor \n @extends {a.Foo} */ a.Bar = function(){}",
"var a = {};\n"
+ "/**\n * @return {undefined}\n * @constructor\n */\n"
+ "a.Foo = function() {\n};\n"
+ "/**\n * @return {undefined}\n * @extends {a.Foo}\n"
+ " * @constructor\n */\n"
+ "a.Bar = function() {\n};\n");
}
public void testTypeAnnotationsInterface() {
assertTypeAnnotations("var a = {};"
+ "/** @interface */ a.Foo = function(){};"
+ "/** @interface \n @extends {a.Foo} */ a.Bar = function(){}",
"var a = {};\n"
+ "/**\n * @interface\n */\n"
+ "a.Foo = function() {\n};\n"
+ "/**\n * @extends {a.Foo}\n"
+ " * @interface\n */\n"
+ "a.Bar = function() {\n};\n");
}
public void testTypeAnnotationsMultipleInterface() {
assertTypeAnnotations("var a = {};"
+ "/** @interface */ a.Foo1 = function(){};"
+ "/** @interface */ a.Foo2 = function(){};"
+ "/** @interface \n @extends {a.Foo1} \n @extends {a.Foo2} */"
+ "a.Bar = function(){}",
"var a = {};\n"
+ "/**\n * @interface\n */\n"
+ "a.Foo1 = function() {\n};\n"
+ "/**\n * @interface\n */\n"
+ "a.Foo2 = function() {\n};\n"
+ "/**\n * @extends {a.Foo1}\n"
+ " * @extends {a.Foo2}\n"
+ " * @interface\n */\n"
+ "a.Bar = function() {\n};\n");
}
public void testTypeAnnotationsMember() {
assertTypeAnnotations("var a = {};"
+ "/** @constructor */ a.Foo = function(){}"
+ "/** @param {string} foo\n"
+ " * @return {number} */\n"
+ "a.Foo.prototype.foo = function(foo) { return 3; };"
+ "/** @type {string|undefined} */"
+ "a.Foo.prototype.bar = '';",
"var a = {};\n"
+ "/**\n * @return {undefined}\n * @constructor\n */\n"
+ "a.Foo = function() {\n};\n"
+ "/**\n"
+ " * @param {string} foo\n"
+ " * @return {number}\n"
+ " */\n"
+ "a.Foo.prototype.foo = function(foo) {\n return 3\n};\n"
+ "/** @type {string} */\n"
+ "a.Foo.prototype.bar = \"\";\n");
}
public void testTypeAnnotationsImplements() {
assertTypeAnnotations("var a = {};"
+ "/** @constructor */ a.Foo = function(){};\n"
+ "/** @interface */ a.I = function(){};\n"
+ "/** @interface */ a.I2 = function(){};\n"
+ "/** @constructor \n @extends {a.Foo}\n"
+ " * @implements {a.I} \n @implements {a.I2}\n"
+ "*/ a.Bar = function(){}",
"var a = {};\n"
+ "/**\n * @return {undefined}\n * @constructor\n */\n"
+ "a.Foo = function() {\n};\n"
+ "/**\n * @interface\n */\n"
+ "a.I = function() {\n};\n"
+ "/**\n * @interface\n */\n"
+ "a.I2 = function() {\n};\n"
+ "/**\n * @return {undefined}\n * @extends {a.Foo}\n"
+ " * @implements {a.I}\n"
+ " * @implements {a.I2}\n * @constructor\n */\n"
+ "a.Bar = function() {\n};\n");
}
public void testTypeAnnotationsDispatcher1() {
assertTypeAnnotations(
"var a = {};\n" +
"/** \n" +
" * @constructor \n" +
" * @javadispatch \n" +
" */\n" +
"a.Foo = function(){}",
"var a = {};\n" +
"/**\n" +
" * @return {undefined}\n" +
" * @constructor\n" +
" * @javadispatch\n" +
" */\n" +
"a.Foo = function() {\n" +
"};\n");
}
public void testTypeAnnotationsDispatcher2() {
assertTypeAnnotations(
"var a = {};\n" +
"/** \n" +
" * @constructor \n" +
" */\n" +
"a.Foo = function(){}\n" +
"/**\n" +
" * @javadispatch\n" +
" */\n" +
"a.Foo.prototype.foo = function() {};",
"var a = {};\n" +
"/**\n" +
" * @return {undefined}\n" +
" * @constructor\n" +
" */\n" +
"a.Foo = function() {\n" +
"};\n" +
"/**\n" +
" * @return {undefined}\n" +
" * @javadispatch\n" +
" */\n" +
"a.Foo.prototype.foo = function() {\n" +
"};\n");
}
public void testU2UFunctionTypeAnnotation() {
assertTypeAnnotations(
"/** @type {!Function} */ var x = function() {}",
"/**\n * @constructor\n */\nvar x = function() {\n};\n");
}
public void testEmitUnknownParamTypesAsAllType() {
assertTypeAnnotations(
"var a = function(x) {}",
"/**\n" +
" * @param {*} x\n" +
" * @return {undefined}\n" +
" */\n" +
"var a = function(x) {\n};\n");
}
public void testOptionalTypesAnnotation() {
assertTypeAnnotations(
"/**\n" +
" * @param {string=} x \n" +
" */\n" +
"var a = function(x) {}",
"/**\n" +
" * @param {string=} x\n" +
" * @return {undefined}\n" +
" */\n" +
"var a = function(x) {\n};\n");
}
public void testVariableArgumentsTypesAnnotation() {
assertTypeAnnotations(
"/**\n" +
" * @param {...string} x \n" +
" */\n" +
"var a = function(x) {}",
"/**\n" +
" * @param {...string} x\n" +
" * @return {undefined}\n" +
" */\n" +
"var a = function(x) {\n};\n");
}
public void testTempConstructor() {
assertTypeAnnotations(
"var x = function() {\n/**\n * @constructor\n */\nfunction t1() {}\n" +
" /**\n * @constructor\n */\nfunction t2() {}\n" +
" t1.prototype = t2.prototype}",
"/**\n * @return {undefined}\n */\nvar x = function() {\n" +
" /**\n * @return {undefined}\n * @constructor\n */\n" +
"function t1() {\n }\n" +
" /**\n * @return {undefined}\n * @constructor\n */\n" +
"function t2() {\n }\n" +
" t1.prototype = t2.prototype\n};\n"
);
}
private void assertPrettyPrint(String js, String expected) {
assertEquals(expected,
parsePrint(js, true, false,
CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD));
}
private void assertTypeAnnotations(String js, String expected) {
assertEquals(expected,
parsePrint(js, true, false,
CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD, true));
}
public void testSubtraction() {
Compiler compiler = new Compiler();
Node n = compiler.parseTestCode("x - -4");
assertEquals(0, compiler.getErrorCount());
assertEquals(
"x- -4",
new CodePrinter.Builder(n).setLineLengthThreshold(
CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD).build());
}
public void testFunctionWithCall() {
assertPrint(
"var user = new function() {"
+ "alert(\"foo\")}",
"var user=new function(){"
+ "alert(\"foo\")}");
assertPrint(
"var user = new function() {"
+ "this.name = \"foo\";"
+ "this.local = function(){alert(this.name)};}",
"var user=new function(){"
+ "this.name=\"foo\";"
+ "this.local=function(){alert(this.name)}}");
}
public void testLineLength() {
// list
assertLineLength("var aba,bcb,cdc",
"var aba,bcb," +
"\ncdc");
// operators, and two breaks
assertLineLength(
"\"foo\"+\"bar,baz,bomb\"+\"whee\"+\";long-string\"\n+\"aaa\"",
"\"foo\"+\"bar,baz,bomb\"+" +
"\n\"whee\"+\";long-string\"+" +
"\n\"aaa\"");
// assignment
assertLineLength("var abazaba=1234",
"var abazaba=" +
"\n1234");
// statements
assertLineLength("var abab=1;var bab=2",
"var abab=1;" +
"\nvar bab=2");
// don't break regexes
assertLineLength("var a=/some[reg](ex),with.*we?rd|chars/i;var b=a",
"var a=/some[reg](ex),with.*we?rd|chars/i;" +
"\nvar b=a");
// don't break strings
assertLineLength("var a=\"foo,{bar};baz\";var b=a",
"var a=\"foo,{bar};baz\";" +
"\nvar b=a");
// don't break before post inc/dec
assertLineLength("var a=\"a\";a++;var b=\"bbb\";",
"var a=\"a\";a++;\n" +
"var b=\"bbb\"");
}
private void assertLineLength(String js, String expected) {
assertEquals(expected,
parsePrint(js, false, true, 10));
}
public void testParsePrintParse() {
testReparse("3;");
testReparse("var a = b;");
testReparse("var x, y, z;");
testReparse("try { foo() } catch(e) { bar() }");
testReparse("try { foo() } catch(e) { bar() } finally { stuff() }");
testReparse("try { foo() } finally { stuff() }");
testReparse("throw 'me'");
testReparse("function foo(a) { return a + 4; }");
testReparse("function foo() { return; }");
testReparse("var a = function(a, b) { foo(); return a + b; }");
testReparse("b = [3, 4, 'paul', \"Buchhe it\",,5];");
testReparse("v = (5, 6, 7, 8)");
testReparse("d = 34.0; x = 0; y = .3; z = -22");
testReparse("d = -x; t = !x + ~y;");
testReparse("'hi'; /* just a test */ stuff(a,b) \n" +
" foo(); // and another \n" +
" bar();");
testReparse("a = b++ + ++c; a = b++-++c; a = - --b; a = - ++b;");
testReparse("a++; b= a++; b = ++a; b = a--; b = --a; a+=2; b-=5");
testReparse("a = (2 + 3) * 4;");
testReparse("a = 1 + (2 + 3) + 4;");
testReparse("x = a ? b : c; x = a ? (b,3,5) : (foo(),bar());");
testReparse("a = b | c || d ^ e " +
"&& f & !g != h << i <= j < k >>> l > m * n % !o");
testReparse("a == b; a != b; a === b; a == b == a;" +
" (a == b) == a; a == (b == a);");
testReparse("if (a > b) a = b; if (b < 3) a = 3; else c = 4;");
testReparse("if (a == b) { a++; } if (a == 0) { a++; } else { a --; }");
testReparse("for (var i in a) b += i;");
testReparse("for (var i = 0; i < 10; i++){ b /= 2;" +
" if (b == 2)break;else continue;}");
testReparse("for (x = 0; x < 10; x++) a /= 2;");
testReparse("for (;;) a++;");
testReparse("while(true) { blah(); }while(true) blah();");
testReparse("do stuff(); while(a>b);");
testReparse("[0, null, , true, false, this];");
testReparse("s.replace(/absc/, 'X').replace(/ab/gi, 'Y');");
testReparse("new Foo; new Bar(a, b,c);");
testReparse("with(foo()) { x = z; y = t; } with(bar()) a = z;");
testReparse("delete foo['bar']; delete foo;");
testReparse("var x = { 'a':'paul', 1:'3', 2:(3,4) };");
testReparse("switch(a) { case 2: case 3: stuff(); break;" +
"case 4: morestuff(); break; default: done();}");
testReparse("x = foo['bar'] + foo['my stuff'] + foo[bar] + f.stuff;");
testReparse("a.v = b.v; x['foo'] = y['zoo'];");
testReparse("'test' in x; 3 in x; a in x;");
testReparse("'foo\"bar' + \"foo'c\" + 'stuff\\n and \\\\more'");
testReparse("x.__proto__;");
}
private void testReparse(String code) {
Compiler compiler = new Compiler();
Node parse1 = parse(code);
Node parse2 = parse(new CodePrinter.Builder(parse1).build());
String explanation = parse1.checkTreeEquals(parse2);
assertNull("\nExpected: " + compiler.toSource(parse1) +
"\nResult: " + compiler.toSource(parse2) +
"\n" + explanation, explanation);
}
public void testDoLoopIECompatiblity() {
// Do loops within IFs cause syntax errors in IE6 and IE7.
assertPrint("function f(){if(e1){do foo();while(e2)}else foo()}",
"function f(){if(e1){do foo();while(e2)}else foo()}");
assertPrint("function f(){if(e1)do foo();while(e2)else foo()}",
"function f(){if(e1){do foo();while(e2)}else foo()}");
assertPrint("if(x){do{foo()}while(y)}else bar()",
"if(x){do foo();while(y)}else bar()");
assertPrint("if(x)do{foo()}while(y);else bar()",
"if(x){do foo();while(y)}else bar()");
assertPrint("if(x){do{foo()}while(y)}",
"if(x){do foo();while(y)}");
assertPrint("if(x)do{foo()}while(y);",
"if(x){do foo();while(y)}");
assertPrint("if(x)A:do{foo()}while(y);",
"if(x){A:do foo();while(y)}");
assertPrint("var i = 0;a: do{b: do{i++;break b;} while(0);} while(0);",
"var i=0;a:do{b:do{i++;break b}while(0)}while(0)");
}
public void testFunctionSafariCompatiblity() {
// Functions within IFs cause syntax errors on Safari.
assertPrint("function f(){if(e1){function goo(){return true}}else foo()}",
"function f(){if(e1){function goo(){return true}}else foo()}");
assertPrint("function f(){if(e1)function goo(){return true}else foo()}",
"function f(){if(e1){function goo(){return true}}else foo()}");
assertPrint("if(e1){function goo(){return true}}",
"if(e1){function goo(){return true}}");
assertPrint("if(e1)function goo(){return true}",
"if(e1){function goo(){return true}}");
assertPrint("if(e1)A:function goo(){return true}",
"if(e1){A:function goo(){return true}}");
}
public void testExponents() {
assertPrintNumber("1", 1);
assertPrintNumber("10", 10);
assertPrintNumber("100", 100);
assertPrintNumber("1E3", 1000);
assertPrintNumber("1E4", 10000);
assertPrintNumber("1E5", 100000);
assertPrintNumber("-1", -1);
assertPrintNumber("-10", -10);
assertPrintNumber("-100", -100);
assertPrintNumber("-1E3", -1000);
assertPrintNumber("-12341234E4", -123412340000L);
assertPrintNumber("1E18", 1000000000000000000L);
assertPrintNumber("1E5", 100000.0);
assertPrintNumber("100000.1", 100000.1);
assertPrintNumber("1.0E-6", 0.000001);
}
// Make sure to test as both a String and a Node, because
// negative numbers do not parse consistently from strings.
private void assertPrintNumber(String expected, double number) {
assertPrint(String.valueOf(number), expected);
assertPrintNode(expected, Node.newNumber(number));
}
private void assertPrintNumber(String expected, int number) {
assertPrint(String.valueOf(number), expected);
assertPrintNode(expected, Node.newNumber(number));
}
public void testDirectEval() {
assertPrint("eval('1');", "eval(\"1\")");
}
public void testIndirectEval() {
Node n = parse("eval('1');");
assertPrintNode("eval(\"1\")", n);
n.getFirstChild().getFirstChild().getFirstChild().putBooleanProp(
Node.DIRECT_EVAL, false);
assertPrintNode("(0,eval)(\"1\")", n);
}
public void testFreeCall1() {
assertPrint("foo(a);", "foo(a)");
assertPrint("x.foo(a);", "x.foo(a)");
}
public void testFreeCall2() {
Node n = parse("foo(a);");
assertPrintNode("foo(a)", n);
Node call = n.getFirstChild().getFirstChild();
assertTrue(call.getType() == Token.CALL);
call.putBooleanProp(Node.FREE_CALL, true);
assertPrintNode("foo(a)", n);
}
public void testFreeCall3() {
Node n = parse("x.foo(a);");
assertPrintNode("x.foo(a)", n);
Node call = n.getFirstChild().getFirstChild();
assertTrue(call.getType() == Token.CALL);
call.putBooleanProp(Node.FREE_CALL, true);
assertPrintNode("(0,x.foo)(a)", n);
}
public void testPrintScript() {
// Verify that SCRIPT nodes not marked as synthetic are printed as
// blocks.
Node ast = new Node(Token.SCRIPT,
new Node(Token.EXPR_RESULT, Node.newString("f")),
new Node(Token.EXPR_RESULT, Node.newString("g")));
String result = new CodePrinter.Builder(ast).setPrettyPrint(true).build();
assertEquals("\"f\";\n\"g\";\n", result);
}
public void testObjectLit() {
assertPrint("({x:1})", "({x:1})");
assertPrint("var x=({x:1})", "var x={x:1}");
assertPrint("var x={'x':1}", "var x={\"x\":1}");
assertPrint("var x={1:1}", "var x={1:1}");
}
public void testObjectLit2() {
assertPrint("var x={1:1}", "var x={1:1}");
assertPrint("var x={'1':1}", "var x={1:1}");
assertPrint("var x={'1.0':1}", "var x={\"1.0\":1}");
assertPrint("var x={1.5:1}", "var x={\"1.5\":1}");
}
public void testObjectLit3() {
assertPrint("var x={3E9:1}",
"var x={3E9:1}");
assertPrint("var x={'3000000000':1}", // More than 31 bits
"var x={3E9:1}");
assertPrint("var x={'3000000001':1}",
"var x={3000000001:1}");
assertPrint("var x={'6000000001':1}", // More than 32 bits
"var x={6000000001:1}");
assertPrint("var x={\"12345678901234567\":1}", // More than 53 bits
"var x={\"12345678901234567\":1}");
}
public void testObjectLit4() {
// More than 128 bits.
assertPrint(
"var x={\"123456789012345671234567890123456712345678901234567\":1}",
"var x={\"123456789012345671234567890123456712345678901234567\":1}");
}
public void testGetter() {
assertPrint("var x = {}", "var x={}");
assertPrint("var x = {get a() {return 1}}", "var x={get a(){return 1}}");
assertPrint(
"var x = {get a() {}, get b(){}}",
"var x={get a(){},get b(){}}");
assertPrint(
"var x = {get 'a'() {return 1}}",
"var x={get \"a\"(){return 1}}");
assertPrint(
"var x = {get 1() {return 1}}",
"var x={get 1(){return 1}}");
assertPrint(
"var x = {get \"()\"() {return 1}}",
"var x={get \"()\"(){return 1}}");
}
public void testSetter() {
assertPrint("var x = {}", "var x={}");
assertPrint(
"var x = {set a(y) {return 1}}",
"var x={set a(y){return 1}}");
assertPrint(
"var x = {get 'a'() {return 1}}",
"var x={get \"a\"(){return 1}}");
assertPrint(
"var x = {set 1(y) {return 1}}",
"var x={set 1(y){return 1}}");
assertPrint(
"var x = {set \"(x)\"(y) {return 1}}",
"var x={set \"(x)\"(y){return 1}}");
}
public void testNegCollapse() {
// Collapse the negative symbol on numbers at generation time,
// to match the Rhino behavior.
assertPrint("var x = - - 2;", "var x=2");
assertPrint("var x = - (2);", "var x=-2");
}
public void testStrict() {
String result = parsePrint("var x", false, false, 0, false, true);
assertEquals("'use strict';var x", result);
}
public void testArrayLiteral() {
assertPrint("var x = [,];","var x=[,]");
assertPrint("var x = [,,];","var x=[,,]");
assertPrint("var x = [,s,,];","var x=[,s,,]");
assertPrint("var x = [,s];","var x=[,s]");
assertPrint("var x = [s,];","var x=[s]");
}
public void testZero() {
assertPrint("var x ='\\0';", "var x=\"\\000\"");
assertPrint("var x ='\\x00';", "var x=\"\\000\"");
assertPrint("var x ='\\u0000';", "var x=\"\\000\"");
assertPrint("var x ='\\u00003';", "var x=\"\\0003\"");
}
public void testUnicode() {
assertPrint("var x ='\\x0f';", "var x=\"\\u000f\"");
assertPrint("var x ='\\x68';", "var x=\"h\"");
assertPrint("var x ='\\x7f';", "var x=\"\\u007f\"");
}
} |
||
public void testSingleOptionSingleArgument() throws Exception {
Parser parser = createDefaultValueParser(new String[]{"100", "1000"});
String enteredValue1 = "1";
String[] args = new String[]{"-b", enteredValue1};
CommandLine cl = parser.parse(args);
CommandLine cmd = cl;
assertNotNull(cmd);
List b = cmd.getValues("-b");
assertEquals("[" + enteredValue1 + ", 1000]", b + "");
} | org.apache.commons.cli2.bug.BugCLI158Test::testSingleOptionSingleArgument | src/test/org/apache/commons/cli2/bug/BugCLI158Test.java | 70 | src/test/org/apache/commons/cli2/bug/BugCLI158Test.java | testSingleOptionSingleArgument | /**
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.cli2.bug;
import java.util.Arrays;
import java.util.List;
import junit.framework.TestCase;
import org.apache.commons.cli2.CommandLine;
import org.apache.commons.cli2.Group;
import org.apache.commons.cli2.builder.ArgumentBuilder;
import org.apache.commons.cli2.builder.DefaultOptionBuilder;
import org.apache.commons.cli2.builder.GroupBuilder;
import org.apache.commons.cli2.commandline.Parser;
import org.apache.commons.cli2.option.DefaultOption;
/**
* http://issues.apache.org/jira/browse/CLI-158
*/
public class BugCLI158Test extends TestCase {
private Parser createDefaultValueParser(String[] defaults) {
final DefaultOptionBuilder obuilder = new DefaultOptionBuilder();
final ArgumentBuilder abuilder = new ArgumentBuilder();
final GroupBuilder gbuilder = new GroupBuilder();
DefaultOption bOption = obuilder.withShortName("b")
.withLongName("b")
.withArgument(abuilder.withName("b")
.withMinimum(0)
.withMaximum(defaults.length)
.withDefaults(Arrays.asList(defaults))
.create())
.create();
Group options = gbuilder
.withName("options")
.withOption(bOption)
.create();
Parser parser = new Parser();
parser.setHelpTrigger("--help");
parser.setGroup(options);
return parser;
}
public void testSingleOptionSingleArgument() throws Exception {
Parser parser = createDefaultValueParser(new String[]{"100", "1000"});
String enteredValue1 = "1";
String[] args = new String[]{"-b", enteredValue1};
CommandLine cl = parser.parse(args);
CommandLine cmd = cl;
assertNotNull(cmd);
List b = cmd.getValues("-b");
assertEquals("[" + enteredValue1 + ", 1000]", b + "");
}
public void testSingleOptionNoArgument() throws Exception {
Parser parser = createDefaultValueParser(new String[]{"100", "1000"});
String[] args = new String[]{"-b"};
CommandLine cl = parser.parse(args);
CommandLine cmd = cl;
assertNotNull(cmd);
List b = cmd.getValues("-b");
assertEquals("[100, 1000]", b + "");
}
public void testSingleOptionMaximumNumberOfArgument() throws Exception {
String[] args = new String[]{"-b", "1", "2"};
final ArgumentBuilder abuilder = new ArgumentBuilder();
final DefaultOptionBuilder obuilder = new DefaultOptionBuilder();
final GroupBuilder gbuilder = new GroupBuilder();
DefaultOption bOption = obuilder.withShortName("b")
.withLongName("b")
.withArgument(abuilder.withName("b")
.withMinimum(2)
.withMaximum(4)
.withDefault("100")
.withDefault("1000")
.withDefault("10000")
.create())
.create();
Group options = gbuilder
.withName("options")
.withOption(bOption)
.create();
Parser parser = new Parser();
parser.setHelpTrigger("--help");
parser.setGroup(options);
CommandLine cl = parser.parse(args);
CommandLine cmd = cl;
assertNotNull(cmd);
List b = cmd.getValues("-b");
assertEquals("[1, 2, 10000]", b + "");
}
} | // You are a professional Java test case writer, please create a test case named `testSingleOptionSingleArgument` for the issue `Cli-CLI-158`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Cli-CLI-158
//
// ## Issue-Title:
// deafult arguments only works if no arguments are submitted
//
// ## Issue-Description:
//
// When using multple arguments and defaults, the behaviour is counter-intuitive and will only pick up a default if no args are passed in.
//
//
// For instance in the code below I have set up so 0, 1, or 2 args may bve accepted, with defaults 100 and 1000.
//
//
// I expect it to behave as follows.
//
// 1. for 2 args, 1 and 2 the values should be 1 and 2. This works as expected.
//
// 2. for 0 args passed in the values should be 100 and 1000, picking up both of the defaults. This works as expected
//
//
// 3. for 1 arg passed in the values should be 1 and 1000, so the second argument picks up the second default value. The valuse become just 1, which is not as expected..
//
//
// Currently, in the second case will only return 1 and ignore the defaults.
//
//
// public void testSingleOptionSingleArgument() throws Exception {
//
// String defaulValue1 = "100";
//
// String defaultValue2 = "1000";
//
// final DefaultOptionBuilder obuilder = new DefaultOptionBuilder();
//
// final ArgumentBuilder abuilder = new ArgumentBuilder();
//
// final GroupBuilder gbuilder = new GroupBuilder();
//
//
// DefaultOption bOption = obuilder.withShortName("b")
//
// .withLongName("b")
//
// .withArgument(abuilder.withName("b")
//
// .withMinimum(0)
//
// .withMaximum(2)
//
// .withDefault(defaulValue1)
//
// .withDefault(defaultValue2)
//
// .create())
//
// .create();
//
//
// Group options = gbuilder
//
// .withName("options")
//
// .withOption(bOption)
//
// .create();
//
//
// Parser parser = new Parser();
//
// parser.setHelpTrigger("--help");
//
// parser.setGroup(options);
//
// String enteredValue1 = "1";
//
// String[] args = new String[]
//
//
// {"-b", enteredValue1}
// ;
//
// CommandLine cl = parser.parse(args);
//
// CommandLine cmd = cl;
//
// assertNotNull(cmd);
//
// List b = cmd.getValues("-b");
//
// assertEquals("[" + enteredValue1 + "]", b + "");
//
// }
//
//
//
//
//
public void testSingleOptionSingleArgument() throws Exception {
| 70 | 15 | 61 | src/test/org/apache/commons/cli2/bug/BugCLI158Test.java | src/test | ```markdown
## Issue-ID: Cli-CLI-158
## Issue-Title:
deafult arguments only works if no arguments are submitted
## Issue-Description:
When using multple arguments and defaults, the behaviour is counter-intuitive and will only pick up a default if no args are passed in.
For instance in the code below I have set up so 0, 1, or 2 args may bve accepted, with defaults 100 and 1000.
I expect it to behave as follows.
1. for 2 args, 1 and 2 the values should be 1 and 2. This works as expected.
2. for 0 args passed in the values should be 100 and 1000, picking up both of the defaults. This works as expected
3. for 1 arg passed in the values should be 1 and 1000, so the second argument picks up the second default value. The valuse become just 1, which is not as expected..
Currently, in the second case will only return 1 and ignore the defaults.
public void testSingleOptionSingleArgument() throws Exception {
String defaulValue1 = "100";
String defaultValue2 = "1000";
final DefaultOptionBuilder obuilder = new DefaultOptionBuilder();
final ArgumentBuilder abuilder = new ArgumentBuilder();
final GroupBuilder gbuilder = new GroupBuilder();
DefaultOption bOption = obuilder.withShortName("b")
.withLongName("b")
.withArgument(abuilder.withName("b")
.withMinimum(0)
.withMaximum(2)
.withDefault(defaulValue1)
.withDefault(defaultValue2)
.create())
.create();
Group options = gbuilder
.withName("options")
.withOption(bOption)
.create();
Parser parser = new Parser();
parser.setHelpTrigger("--help");
parser.setGroup(options);
String enteredValue1 = "1";
String[] args = new String[]
{"-b", enteredValue1}
;
CommandLine cl = parser.parse(args);
CommandLine cmd = cl;
assertNotNull(cmd);
List b = cmd.getValues("-b");
assertEquals("[" + enteredValue1 + "]", b + "");
}
```
You are a professional Java test case writer, please create a test case named `testSingleOptionSingleArgument` for the issue `Cli-CLI-158`, utilizing the provided issue report information and the following function signature.
```java
public void testSingleOptionSingleArgument() throws Exception {
```
| 61 | [
"org.apache.commons.cli2.commandline.WriteableCommandLineImpl"
] | a17f5954f3df8a9aa54b59d8338353946b37489cc0b6e9059a1fca9dcdb566b8 | public void testSingleOptionSingleArgument() throws Exception | // You are a professional Java test case writer, please create a test case named `testSingleOptionSingleArgument` for the issue `Cli-CLI-158`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Cli-CLI-158
//
// ## Issue-Title:
// deafult arguments only works if no arguments are submitted
//
// ## Issue-Description:
//
// When using multple arguments and defaults, the behaviour is counter-intuitive and will only pick up a default if no args are passed in.
//
//
// For instance in the code below I have set up so 0, 1, or 2 args may bve accepted, with defaults 100 and 1000.
//
//
// I expect it to behave as follows.
//
// 1. for 2 args, 1 and 2 the values should be 1 and 2. This works as expected.
//
// 2. for 0 args passed in the values should be 100 and 1000, picking up both of the defaults. This works as expected
//
//
// 3. for 1 arg passed in the values should be 1 and 1000, so the second argument picks up the second default value. The valuse become just 1, which is not as expected..
//
//
// Currently, in the second case will only return 1 and ignore the defaults.
//
//
// public void testSingleOptionSingleArgument() throws Exception {
//
// String defaulValue1 = "100";
//
// String defaultValue2 = "1000";
//
// final DefaultOptionBuilder obuilder = new DefaultOptionBuilder();
//
// final ArgumentBuilder abuilder = new ArgumentBuilder();
//
// final GroupBuilder gbuilder = new GroupBuilder();
//
//
// DefaultOption bOption = obuilder.withShortName("b")
//
// .withLongName("b")
//
// .withArgument(abuilder.withName("b")
//
// .withMinimum(0)
//
// .withMaximum(2)
//
// .withDefault(defaulValue1)
//
// .withDefault(defaultValue2)
//
// .create())
//
// .create();
//
//
// Group options = gbuilder
//
// .withName("options")
//
// .withOption(bOption)
//
// .create();
//
//
// Parser parser = new Parser();
//
// parser.setHelpTrigger("--help");
//
// parser.setGroup(options);
//
// String enteredValue1 = "1";
//
// String[] args = new String[]
//
//
// {"-b", enteredValue1}
// ;
//
// CommandLine cl = parser.parse(args);
//
// CommandLine cmd = cl;
//
// assertNotNull(cmd);
//
// List b = cmd.getValues("-b");
//
// assertEquals("[" + enteredValue1 + "]", b + "");
//
// }
//
//
//
//
//
| Cli | /**
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.cli2.bug;
import java.util.Arrays;
import java.util.List;
import junit.framework.TestCase;
import org.apache.commons.cli2.CommandLine;
import org.apache.commons.cli2.Group;
import org.apache.commons.cli2.builder.ArgumentBuilder;
import org.apache.commons.cli2.builder.DefaultOptionBuilder;
import org.apache.commons.cli2.builder.GroupBuilder;
import org.apache.commons.cli2.commandline.Parser;
import org.apache.commons.cli2.option.DefaultOption;
/**
* http://issues.apache.org/jira/browse/CLI-158
*/
public class BugCLI158Test extends TestCase {
private Parser createDefaultValueParser(String[] defaults) {
final DefaultOptionBuilder obuilder = new DefaultOptionBuilder();
final ArgumentBuilder abuilder = new ArgumentBuilder();
final GroupBuilder gbuilder = new GroupBuilder();
DefaultOption bOption = obuilder.withShortName("b")
.withLongName("b")
.withArgument(abuilder.withName("b")
.withMinimum(0)
.withMaximum(defaults.length)
.withDefaults(Arrays.asList(defaults))
.create())
.create();
Group options = gbuilder
.withName("options")
.withOption(bOption)
.create();
Parser parser = new Parser();
parser.setHelpTrigger("--help");
parser.setGroup(options);
return parser;
}
public void testSingleOptionSingleArgument() throws Exception {
Parser parser = createDefaultValueParser(new String[]{"100", "1000"});
String enteredValue1 = "1";
String[] args = new String[]{"-b", enteredValue1};
CommandLine cl = parser.parse(args);
CommandLine cmd = cl;
assertNotNull(cmd);
List b = cmd.getValues("-b");
assertEquals("[" + enteredValue1 + ", 1000]", b + "");
}
public void testSingleOptionNoArgument() throws Exception {
Parser parser = createDefaultValueParser(new String[]{"100", "1000"});
String[] args = new String[]{"-b"};
CommandLine cl = parser.parse(args);
CommandLine cmd = cl;
assertNotNull(cmd);
List b = cmd.getValues("-b");
assertEquals("[100, 1000]", b + "");
}
public void testSingleOptionMaximumNumberOfArgument() throws Exception {
String[] args = new String[]{"-b", "1", "2"};
final ArgumentBuilder abuilder = new ArgumentBuilder();
final DefaultOptionBuilder obuilder = new DefaultOptionBuilder();
final GroupBuilder gbuilder = new GroupBuilder();
DefaultOption bOption = obuilder.withShortName("b")
.withLongName("b")
.withArgument(abuilder.withName("b")
.withMinimum(2)
.withMaximum(4)
.withDefault("100")
.withDefault("1000")
.withDefault("10000")
.create())
.create();
Group options = gbuilder
.withName("options")
.withOption(bOption)
.create();
Parser parser = new Parser();
parser.setHelpTrigger("--help");
parser.setGroup(options);
CommandLine cl = parser.parse(args);
CommandLine cmd = cl;
assertNotNull(cmd);
List b = cmd.getValues("-b");
assertEquals("[1, 2, 10000]", b + "");
}
} |
||
public void testDelegateWithTokenBuffer() throws Exception
{
ObjectMapper mapper = new ObjectMapper();
Value592 value = mapper.readValue("{\"a\":1,\"b\":2}", Value592.class);
assertNotNull(value);
Object ob = value.stuff;
assertEquals(TokenBuffer.class, ob.getClass());
JsonParser jp = ((TokenBuffer) ob).asParser();
assertToken(JsonToken.START_OBJECT, jp.nextToken());
assertToken(JsonToken.FIELD_NAME, jp.nextToken());
assertEquals("a", jp.getCurrentName());
assertToken(JsonToken.VALUE_NUMBER_INT, jp.nextToken());
assertEquals(1, jp.getIntValue());
assertToken(JsonToken.FIELD_NAME, jp.nextToken());
assertEquals("b", jp.getCurrentName());
assertToken(JsonToken.VALUE_NUMBER_INT, jp.nextToken());
assertEquals(2, jp.getIntValue());
assertToken(JsonToken.END_OBJECT, jp.nextToken());
jp.close();
} | com.fasterxml.jackson.databind.creators.TestCreatorsDelegating::testDelegateWithTokenBuffer | src/test/java/com/fasterxml/jackson/databind/creators/TestCreatorsDelegating.java | 142 | src/test/java/com/fasterxml/jackson/databind/creators/TestCreatorsDelegating.java | testDelegateWithTokenBuffer | package com.fasterxml.jackson.databind.creators;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.core.JsonToken;
import com.fasterxml.jackson.databind.*;
import com.fasterxml.jackson.databind.util.TokenBuffer;
public class TestCreatorsDelegating extends BaseMapTest
{
static class BooleanBean
{
protected Boolean value;
public BooleanBean(Boolean v) { value = v; }
@JsonCreator
protected static BooleanBean create(Boolean value) {
return new BooleanBean(value);
}
}
// for [JACKSON-711]; should allow delegate-based one(s) too
static class CtorBean711
{
protected String name;
protected int age;
@JsonCreator
public CtorBean711(@JacksonInject String n, int a)
{
name = n;
age = a;
}
}
// for [JACKSON-711]; should allow delegate-based one(s) too
static class FactoryBean711
{
protected String name1;
protected String name2;
protected int age;
private FactoryBean711(int a, String n1, String n2) {
age = a;
name1 = n1;
name2 = n2;
}
@JsonCreator
public static FactoryBean711 create(@JacksonInject String n1, int a, @JacksonInject String n2) {
return new FactoryBean711(a, n1, n2);
}
}
static class Value592
{
protected Object stuff;
protected Value592(Object ob, boolean bogus) {
stuff = ob;
}
@JsonCreator
public static Value592 from(TokenBuffer buffer) {
return new Value592(buffer, false);
}
}
/*
/**********************************************************
/* Unit tests
/**********************************************************
*/
public void testBooleanDelegate() throws Exception
{
ObjectMapper m = new ObjectMapper();
// should obviously work with booleans...
BooleanBean bb = m.readValue("true", BooleanBean.class);
assertEquals(Boolean.TRUE, bb.value);
// but also with value conversion from String
bb = m.readValue(quote("true"), BooleanBean.class);
assertEquals(Boolean.TRUE, bb.value);
}
// As per [JACKSON-711]: should also work with delegate model (single non-annotated arg)
public void testWithCtorAndDelegate() throws Exception
{
ObjectMapper mapper = new ObjectMapper();
mapper.setInjectableValues(new InjectableValues.Std()
.addValue(String.class, "Pooka")
);
CtorBean711 bean = null;
try {
bean = mapper.readValue("38", CtorBean711.class);
} catch (JsonMappingException e) {
fail("Did not expect problems, got: "+e.getMessage());
}
assertEquals(38, bean.age);
assertEquals("Pooka", bean.name);
}
public void testWithFactoryAndDelegate() throws Exception
{
ObjectMapper mapper = new ObjectMapper();
mapper.setInjectableValues(new InjectableValues.Std()
.addValue(String.class, "Fygar")
);
FactoryBean711 bean = null;
try {
bean = mapper.readValue("38", FactoryBean711.class);
} catch (JsonMappingException e) {
fail("Did not expect problems, got: "+e.getMessage());
}
assertEquals(38, bean.age);
assertEquals("Fygar", bean.name1);
assertEquals("Fygar", bean.name2);
}
// [databind#592]
public void testDelegateWithTokenBuffer() throws Exception
{
ObjectMapper mapper = new ObjectMapper();
Value592 value = mapper.readValue("{\"a\":1,\"b\":2}", Value592.class);
assertNotNull(value);
Object ob = value.stuff;
assertEquals(TokenBuffer.class, ob.getClass());
JsonParser jp = ((TokenBuffer) ob).asParser();
assertToken(JsonToken.START_OBJECT, jp.nextToken());
assertToken(JsonToken.FIELD_NAME, jp.nextToken());
assertEquals("a", jp.getCurrentName());
assertToken(JsonToken.VALUE_NUMBER_INT, jp.nextToken());
assertEquals(1, jp.getIntValue());
assertToken(JsonToken.FIELD_NAME, jp.nextToken());
assertEquals("b", jp.getCurrentName());
assertToken(JsonToken.VALUE_NUMBER_INT, jp.nextToken());
assertEquals(2, jp.getIntValue());
assertToken(JsonToken.END_OBJECT, jp.nextToken());
jp.close();
}
} | // You are a professional Java test case writer, please create a test case named `testDelegateWithTokenBuffer` for the issue `JacksonDatabind-592`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonDatabind-592
//
// ## Issue-Title:
// Possibly wrong TokenBuffer delegate deserialization using @JsonCreator
//
// ## Issue-Description:
//
// ```
// class Value {
// @JsonCreator
// public static Value from(TokenBuffer buffer) {
// ...
// }
// ```
//
// Given JSON string is `{ "a":1, "b":null }`, it is expected that while deserializing using delegate buffer,
//
// current token will be start object `{`, and rest of the tokens will be available in buffer:
//
//
//
// ```
// [START_OBJECT, FIELD_NAME, VALUE_NUMBER_INT, FIELD_NAME, VALUE_NULL, END_OBJECT]
//
// ```
//
// But, buffers ends up being started with field name and then contains single attribute value
//
//
//
// ```
// [FIELD_NAME, VALUE_NUMBER_INT]
//
// ```
//
// It's due to how `TokenBuffer#copyCurrentStructure` works when we have current token as a `FIELD_NAME`, rather than `START_OBJECT`, because it's forced to move to next token [BeanDeserializer.java:120](https://github.com/FasterXML/jackson-databind/blob/2.4/src/main/java/com/fasterxml/jackson/databind/deser/BeanDeserializer.java#L120)
//
//
// Hope this helps to nail it down. Is it an intended behavior, or it's regression/bug?
//
//
//
//
public void testDelegateWithTokenBuffer() throws Exception {
| 142 | // [databind#592] | 7 | 123 | src/test/java/com/fasterxml/jackson/databind/creators/TestCreatorsDelegating.java | src/test/java | ```markdown
## Issue-ID: JacksonDatabind-592
## Issue-Title:
Possibly wrong TokenBuffer delegate deserialization using @JsonCreator
## Issue-Description:
```
class Value {
@JsonCreator
public static Value from(TokenBuffer buffer) {
...
}
```
Given JSON string is `{ "a":1, "b":null }`, it is expected that while deserializing using delegate buffer,
current token will be start object `{`, and rest of the tokens will be available in buffer:
```
[START_OBJECT, FIELD_NAME, VALUE_NUMBER_INT, FIELD_NAME, VALUE_NULL, END_OBJECT]
```
But, buffers ends up being started with field name and then contains single attribute value
```
[FIELD_NAME, VALUE_NUMBER_INT]
```
It's due to how `TokenBuffer#copyCurrentStructure` works when we have current token as a `FIELD_NAME`, rather than `START_OBJECT`, because it's forced to move to next token [BeanDeserializer.java:120](https://github.com/FasterXML/jackson-databind/blob/2.4/src/main/java/com/fasterxml/jackson/databind/deser/BeanDeserializer.java#L120)
Hope this helps to nail it down. Is it an intended behavior, or it's regression/bug?
```
You are a professional Java test case writer, please create a test case named `testDelegateWithTokenBuffer` for the issue `JacksonDatabind-592`, utilizing the provided issue report information and the following function signature.
```java
public void testDelegateWithTokenBuffer() throws Exception {
```
| 123 | [
"com.fasterxml.jackson.databind.util.TokenBuffer"
] | a2dc0da77d9a80cdcd7b5a6068ba0902b4efe7ab7314f859a4185357694d2eb5 | public void testDelegateWithTokenBuffer() throws Exception
| // You are a professional Java test case writer, please create a test case named `testDelegateWithTokenBuffer` for the issue `JacksonDatabind-592`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonDatabind-592
//
// ## Issue-Title:
// Possibly wrong TokenBuffer delegate deserialization using @JsonCreator
//
// ## Issue-Description:
//
// ```
// class Value {
// @JsonCreator
// public static Value from(TokenBuffer buffer) {
// ...
// }
// ```
//
// Given JSON string is `{ "a":1, "b":null }`, it is expected that while deserializing using delegate buffer,
//
// current token will be start object `{`, and rest of the tokens will be available in buffer:
//
//
//
// ```
// [START_OBJECT, FIELD_NAME, VALUE_NUMBER_INT, FIELD_NAME, VALUE_NULL, END_OBJECT]
//
// ```
//
// But, buffers ends up being started with field name and then contains single attribute value
//
//
//
// ```
// [FIELD_NAME, VALUE_NUMBER_INT]
//
// ```
//
// It's due to how `TokenBuffer#copyCurrentStructure` works when we have current token as a `FIELD_NAME`, rather than `START_OBJECT`, because it's forced to move to next token [BeanDeserializer.java:120](https://github.com/FasterXML/jackson-databind/blob/2.4/src/main/java/com/fasterxml/jackson/databind/deser/BeanDeserializer.java#L120)
//
//
// Hope this helps to nail it down. Is it an intended behavior, or it's regression/bug?
//
//
//
//
| JacksonDatabind | package com.fasterxml.jackson.databind.creators;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.core.JsonToken;
import com.fasterxml.jackson.databind.*;
import com.fasterxml.jackson.databind.util.TokenBuffer;
public class TestCreatorsDelegating extends BaseMapTest
{
static class BooleanBean
{
protected Boolean value;
public BooleanBean(Boolean v) { value = v; }
@JsonCreator
protected static BooleanBean create(Boolean value) {
return new BooleanBean(value);
}
}
// for [JACKSON-711]; should allow delegate-based one(s) too
static class CtorBean711
{
protected String name;
protected int age;
@JsonCreator
public CtorBean711(@JacksonInject String n, int a)
{
name = n;
age = a;
}
}
// for [JACKSON-711]; should allow delegate-based one(s) too
static class FactoryBean711
{
protected String name1;
protected String name2;
protected int age;
private FactoryBean711(int a, String n1, String n2) {
age = a;
name1 = n1;
name2 = n2;
}
@JsonCreator
public static FactoryBean711 create(@JacksonInject String n1, int a, @JacksonInject String n2) {
return new FactoryBean711(a, n1, n2);
}
}
static class Value592
{
protected Object stuff;
protected Value592(Object ob, boolean bogus) {
stuff = ob;
}
@JsonCreator
public static Value592 from(TokenBuffer buffer) {
return new Value592(buffer, false);
}
}
/*
/**********************************************************
/* Unit tests
/**********************************************************
*/
public void testBooleanDelegate() throws Exception
{
ObjectMapper m = new ObjectMapper();
// should obviously work with booleans...
BooleanBean bb = m.readValue("true", BooleanBean.class);
assertEquals(Boolean.TRUE, bb.value);
// but also with value conversion from String
bb = m.readValue(quote("true"), BooleanBean.class);
assertEquals(Boolean.TRUE, bb.value);
}
// As per [JACKSON-711]: should also work with delegate model (single non-annotated arg)
public void testWithCtorAndDelegate() throws Exception
{
ObjectMapper mapper = new ObjectMapper();
mapper.setInjectableValues(new InjectableValues.Std()
.addValue(String.class, "Pooka")
);
CtorBean711 bean = null;
try {
bean = mapper.readValue("38", CtorBean711.class);
} catch (JsonMappingException e) {
fail("Did not expect problems, got: "+e.getMessage());
}
assertEquals(38, bean.age);
assertEquals("Pooka", bean.name);
}
public void testWithFactoryAndDelegate() throws Exception
{
ObjectMapper mapper = new ObjectMapper();
mapper.setInjectableValues(new InjectableValues.Std()
.addValue(String.class, "Fygar")
);
FactoryBean711 bean = null;
try {
bean = mapper.readValue("38", FactoryBean711.class);
} catch (JsonMappingException e) {
fail("Did not expect problems, got: "+e.getMessage());
}
assertEquals(38, bean.age);
assertEquals("Fygar", bean.name1);
assertEquals("Fygar", bean.name2);
}
// [databind#592]
public void testDelegateWithTokenBuffer() throws Exception
{
ObjectMapper mapper = new ObjectMapper();
Value592 value = mapper.readValue("{\"a\":1,\"b\":2}", Value592.class);
assertNotNull(value);
Object ob = value.stuff;
assertEquals(TokenBuffer.class, ob.getClass());
JsonParser jp = ((TokenBuffer) ob).asParser();
assertToken(JsonToken.START_OBJECT, jp.nextToken());
assertToken(JsonToken.FIELD_NAME, jp.nextToken());
assertEquals("a", jp.getCurrentName());
assertToken(JsonToken.VALUE_NUMBER_INT, jp.nextToken());
assertEquals(1, jp.getIntValue());
assertToken(JsonToken.FIELD_NAME, jp.nextToken());
assertEquals("b", jp.getCurrentName());
assertToken(JsonToken.VALUE_NUMBER_INT, jp.nextToken());
assertEquals(2, jp.getIntValue());
assertToken(JsonToken.END_OBJECT, jp.nextToken());
jp.close();
}
} |
|
@Test(expected = IllegalArgumentException.class) public void validatesKeysNotEmpty() {
Attribute attr = new Attribute(" ", "Check");
} | org.jsoup.nodes.AttributeTest::validatesKeysNotEmpty | src/test/java/org/jsoup/nodes/AttributeTest.java | 22 | src/test/java/org/jsoup/nodes/AttributeTest.java | validatesKeysNotEmpty | package org.jsoup.nodes;
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class AttributeTest {
@Test public void html() {
Attribute attr = new Attribute("key", "value &");
assertEquals("key=\"value &\"", attr.html());
assertEquals(attr.html(), attr.toString());
}
@Test public void testWithSupplementaryCharacterInAttributeKeyAndValue() {
String s = new String(Character.toChars(135361));
Attribute attr = new Attribute(s, "A" + s + "B");
assertEquals(s + "=\"A" + s + "B\"", attr.html());
assertEquals(attr.html(), attr.toString());
}
@Test(expected = IllegalArgumentException.class) public void validatesKeysNotEmpty() {
Attribute attr = new Attribute(" ", "Check");
}
@Test(expected = IllegalArgumentException.class) public void validatesKeysNotEmptyViaSet() {
Attribute attr = new Attribute("One", "Check");
attr.setKey(" ");
}
} | // You are a professional Java test case writer, please create a test case named `validatesKeysNotEmpty` for the issue `Jsoup-1159`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Jsoup-1159
//
// ## Issue-Title:
// Attribute.java line 45 variable key scope error, it seems should be "this.key"
//
// ## Issue-Description:
// [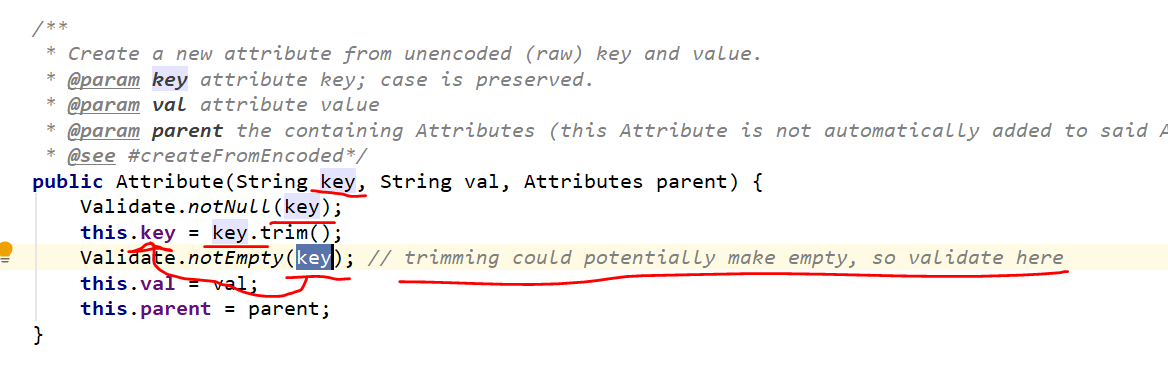](https://user-images.githubusercontent.com/41705526/49982508-ca65db80-ff11-11e8-9833-1775ddcc8871.png)
//
//
// Attribute.java Line 45, it should be:
//
//
//
// ```
// Validate.notEmpty(this.key);
// ```
//
// rather than
//
//
//
// ```
// Validate.notEmpty(key);
// ```
//
// This issue only happens when **key** is blank or empty, in reality this would rarely happen, but in the syntax context it is still an issue, so better fix this.
//
//
//
//
@Test(expected = IllegalArgumentException.class) public void validatesKeysNotEmpty() {
| 22 | 85 | 20 | src/test/java/org/jsoup/nodes/AttributeTest.java | src/test/java | ```markdown
## Issue-ID: Jsoup-1159
## Issue-Title:
Attribute.java line 45 variable key scope error, it seems should be "this.key"
## Issue-Description:
[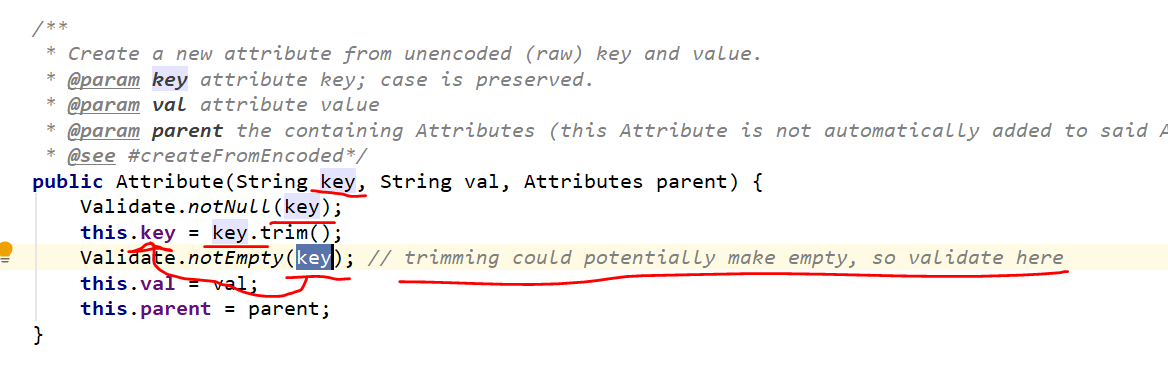](https://user-images.githubusercontent.com/41705526/49982508-ca65db80-ff11-11e8-9833-1775ddcc8871.png)
Attribute.java Line 45, it should be:
```
Validate.notEmpty(this.key);
```
rather than
```
Validate.notEmpty(key);
```
This issue only happens when **key** is blank or empty, in reality this would rarely happen, but in the syntax context it is still an issue, so better fix this.
```
You are a professional Java test case writer, please create a test case named `validatesKeysNotEmpty` for the issue `Jsoup-1159`, utilizing the provided issue report information and the following function signature.
```java
@Test(expected = IllegalArgumentException.class) public void validatesKeysNotEmpty() {
```
| 20 | [
"org.jsoup.nodes.Attribute"
] | a34b8735ff240b9b0dca58e322b76fc0f93f62fc140c4f11bb8dbbfaff171fc1 | @Test(expected = IllegalArgumentException.class) public void validatesKeysNotEmpty() | // You are a professional Java test case writer, please create a test case named `validatesKeysNotEmpty` for the issue `Jsoup-1159`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Jsoup-1159
//
// ## Issue-Title:
// Attribute.java line 45 variable key scope error, it seems should be "this.key"
//
// ## Issue-Description:
// [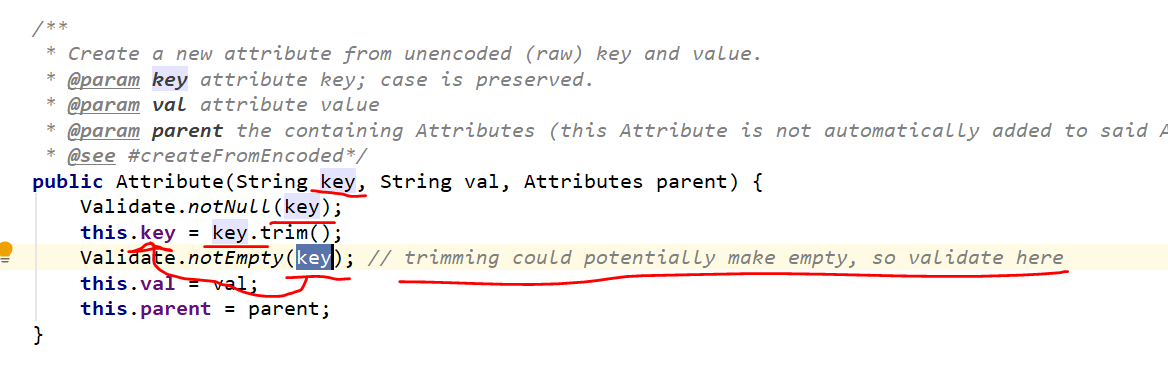](https://user-images.githubusercontent.com/41705526/49982508-ca65db80-ff11-11e8-9833-1775ddcc8871.png)
//
//
// Attribute.java Line 45, it should be:
//
//
//
// ```
// Validate.notEmpty(this.key);
// ```
//
// rather than
//
//
//
// ```
// Validate.notEmpty(key);
// ```
//
// This issue only happens when **key** is blank or empty, in reality this would rarely happen, but in the syntax context it is still an issue, so better fix this.
//
//
//
//
| Jsoup | package org.jsoup.nodes;
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class AttributeTest {
@Test public void html() {
Attribute attr = new Attribute("key", "value &");
assertEquals("key=\"value &\"", attr.html());
assertEquals(attr.html(), attr.toString());
}
@Test public void testWithSupplementaryCharacterInAttributeKeyAndValue() {
String s = new String(Character.toChars(135361));
Attribute attr = new Attribute(s, "A" + s + "B");
assertEquals(s + "=\"A" + s + "B\"", attr.html());
assertEquals(attr.html(), attr.toString());
}
@Test(expected = IllegalArgumentException.class) public void validatesKeysNotEmpty() {
Attribute attr = new Attribute(" ", "Check");
}
@Test(expected = IllegalArgumentException.class) public void validatesKeysNotEmptyViaSet() {
Attribute attr = new Attribute("One", "Check");
attr.setKey(" ");
}
} |
||
public void testInvalidSubClass() throws Exception
{
DefaultPrettyPrinter pp = new MyPrettyPrinter();
try {
pp.createInstance();
fail("Should not pass");
} catch (IllegalStateException e) {
verifyException(e, "does not override");
}
} | com.fasterxml.jackson.core.util.TestDefaultPrettyPrinter::testInvalidSubClass | src/test/java/com/fasterxml/jackson/core/util/TestDefaultPrettyPrinter.java | 172 | src/test/java/com/fasterxml/jackson/core/util/TestDefaultPrettyPrinter.java | testInvalidSubClass | package com.fasterxml.jackson.core.util;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.StringWriter;
import com.fasterxml.jackson.core.*;
public class TestDefaultPrettyPrinter extends BaseTest
{
private final JsonFactory JSON_F = new JsonFactory();
public void testSystemLinefeed() throws IOException
{
PrettyPrinter pp = new DefaultPrettyPrinter();
String LF = System.getProperty("line.separator");
String EXP = "{" + LF +
" \"name\" : \"John Doe\"," + LF +
" \"age\" : 3.14" + LF +
"}";
assertEquals(EXP, _printTestData(pp, false));
assertEquals(EXP, _printTestData(pp, true));
}
public void testWithLineFeed() throws IOException
{
PrettyPrinter pp = new DefaultPrettyPrinter()
.withObjectIndenter(new DefaultIndenter().withLinefeed("\n"));
String EXP = "{\n" +
" \"name\" : \"John Doe\",\n" +
" \"age\" : 3.14\n" +
"}";
assertEquals(EXP, _printTestData(pp, false));
assertEquals(EXP, _printTestData(pp, true));
}
public void testWithIndent() throws IOException
{
PrettyPrinter pp = new DefaultPrettyPrinter()
.withObjectIndenter(new DefaultIndenter().withLinefeed("\n").withIndent(" "));
String EXP = "{\n" +
" \"name\" : \"John Doe\",\n" +
" \"age\" : 3.14\n" +
"}";
assertEquals(EXP, _printTestData(pp, false));
assertEquals(EXP, _printTestData(pp, true));
}
public void testUnixLinefeed() throws IOException
{
PrettyPrinter pp = new DefaultPrettyPrinter()
.withObjectIndenter(new DefaultIndenter(" ", "\n"));
String EXP = "{\n" +
" \"name\" : \"John Doe\",\n" +
" \"age\" : 3.14\n" +
"}";
assertEquals(EXP, _printTestData(pp, false));
assertEquals(EXP, _printTestData(pp, true));
}
public void testWindowsLinefeed() throws IOException
{
PrettyPrinter pp = new DefaultPrettyPrinter()
.withObjectIndenter(new DefaultIndenter(" ", "\r\n"));
String EXP = "{\r\n" +
" \"name\" : \"John Doe\",\r\n" +
" \"age\" : 3.14\r\n" +
"}";
assertEquals(EXP, _printTestData(pp, false));
assertEquals(EXP, _printTestData(pp, true));
}
public void testTabIndent() throws IOException
{
PrettyPrinter pp = new DefaultPrettyPrinter()
.withObjectIndenter(new DefaultIndenter("\t", "\n"));
String EXP = "{\n" +
"\t\"name\" : \"John Doe\",\n" +
"\t\"age\" : 3.14\n" +
"}";
assertEquals(EXP, _printTestData(pp, false));
assertEquals(EXP, _printTestData(pp, true));
}
public void testRootSeparator() throws IOException
{
DefaultPrettyPrinter pp = new DefaultPrettyPrinter()
.withRootSeparator("|");
final String EXP = "1|2|3";
StringWriter sw = new StringWriter();
JsonGenerator gen = JSON_F.createGenerator(sw);
gen.setPrettyPrinter(pp);
gen.writeNumber(1);
gen.writeNumber(2);
gen.writeNumber(3);
gen.close();
assertEquals(EXP, sw.toString());
ByteArrayOutputStream bytes = new ByteArrayOutputStream();
gen = JSON_F.createGenerator(bytes);
gen.setPrettyPrinter(pp);
gen.writeNumber(1);
gen.writeNumber(2);
gen.writeNumber(3);
gen.close();
assertEquals(EXP, bytes.toString("UTF-8"));
// Also: let's try removing separator altogether
pp = pp.withRootSeparator((String) null)
.withArrayIndenter(null)
.withObjectIndenter(null)
.withoutSpacesInObjectEntries();
sw = new StringWriter();
gen = JSON_F.createGenerator(sw);
gen.setPrettyPrinter(pp);
gen.writeNumber(1);
gen.writeStartArray();
gen.writeNumber(2);
gen.writeEndArray();
gen.writeStartObject();
gen.writeFieldName("a");
gen.writeNumber(3);
gen.writeEndObject();
gen.close();
// no root separator, nor array, object
assertEquals("1[2]{\"a\":3}", sw.toString());
}
private String _printTestData(PrettyPrinter pp, boolean useBytes) throws IOException
{
JsonGenerator gen;
StringWriter sw;
ByteArrayOutputStream bytes;
if (useBytes) {
sw = null;
bytes = new ByteArrayOutputStream();
gen = JSON_F.createGenerator(bytes);
} else {
sw = new StringWriter();
bytes = null;
gen = JSON_F.createGenerator(sw);
}
gen.setPrettyPrinter(pp);
gen.writeStartObject();
gen.writeFieldName("name");
gen.writeString("John Doe");
gen.writeFieldName("age");
gen.writeNumber(3.14);
gen.writeEndObject();
gen.close();
if (useBytes) {
return bytes.toString("UTF-8");
}
return sw.toString();
}
// [core#502]: Force sub-classes to reimplement `createInstance`
public void testInvalidSubClass() throws Exception
{
DefaultPrettyPrinter pp = new MyPrettyPrinter();
try {
pp.createInstance();
fail("Should not pass");
} catch (IllegalStateException e) {
verifyException(e, "does not override");
}
}
@SuppressWarnings("serial")
static class MyPrettyPrinter extends DefaultPrettyPrinter { }
} | // You are a professional Java test case writer, please create a test case named `testInvalidSubClass` for the issue `JacksonCore-502`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonCore-502
//
// ## Issue-Title:
// Make DefaultPrettyPrinter.createInstance() to fail for sub-classes
//
// ## Issue-Description:
// Pattern of "blueprint object" (that is, having an instance not used as-is, but that has factory method for creating actual instance) is used by Jackson in couple of places; often for things that implement `Instantiatable`. But one problem is that unless method is left abstract, sub-classing can be problematic -- if sub-class does not override method, then calls will result in an instance of wrong type being created.
//
//
// And this is what can easily happen with `DefaultPrettyPrinter`.
//
//
// A simple solution is for base class to make explicit that if base implementation is called, then instance can not be a sub-class (that is, it is only legal to call on `DefaultPrettyPrinter`, but no sub-class). This is not optimal (ideally check would be done compile-time), but better than getting a mysterious failure.
//
//
//
//
public void testInvalidSubClass() throws Exception {
| 172 | // [core#502]: Force sub-classes to reimplement `createInstance` | 23 | 163 | src/test/java/com/fasterxml/jackson/core/util/TestDefaultPrettyPrinter.java | src/test/java | ```markdown
## Issue-ID: JacksonCore-502
## Issue-Title:
Make DefaultPrettyPrinter.createInstance() to fail for sub-classes
## Issue-Description:
Pattern of "blueprint object" (that is, having an instance not used as-is, but that has factory method for creating actual instance) is used by Jackson in couple of places; often for things that implement `Instantiatable`. But one problem is that unless method is left abstract, sub-classing can be problematic -- if sub-class does not override method, then calls will result in an instance of wrong type being created.
And this is what can easily happen with `DefaultPrettyPrinter`.
A simple solution is for base class to make explicit that if base implementation is called, then instance can not be a sub-class (that is, it is only legal to call on `DefaultPrettyPrinter`, but no sub-class). This is not optimal (ideally check would be done compile-time), but better than getting a mysterious failure.
```
You are a professional Java test case writer, please create a test case named `testInvalidSubClass` for the issue `JacksonCore-502`, utilizing the provided issue report information and the following function signature.
```java
public void testInvalidSubClass() throws Exception {
```
| 163 | [
"com.fasterxml.jackson.core.util.DefaultPrettyPrinter"
] | a350b1a6f5a06c24d159212da7876bc155f274551dee59bca150bf12daf401d8 | public void testInvalidSubClass() throws Exception
| // You are a professional Java test case writer, please create a test case named `testInvalidSubClass` for the issue `JacksonCore-502`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonCore-502
//
// ## Issue-Title:
// Make DefaultPrettyPrinter.createInstance() to fail for sub-classes
//
// ## Issue-Description:
// Pattern of "blueprint object" (that is, having an instance not used as-is, but that has factory method for creating actual instance) is used by Jackson in couple of places; often for things that implement `Instantiatable`. But one problem is that unless method is left abstract, sub-classing can be problematic -- if sub-class does not override method, then calls will result in an instance of wrong type being created.
//
//
// And this is what can easily happen with `DefaultPrettyPrinter`.
//
//
// A simple solution is for base class to make explicit that if base implementation is called, then instance can not be a sub-class (that is, it is only legal to call on `DefaultPrettyPrinter`, but no sub-class). This is not optimal (ideally check would be done compile-time), but better than getting a mysterious failure.
//
//
//
//
| JacksonCore | package com.fasterxml.jackson.core.util;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.StringWriter;
import com.fasterxml.jackson.core.*;
public class TestDefaultPrettyPrinter extends BaseTest
{
private final JsonFactory JSON_F = new JsonFactory();
public void testSystemLinefeed() throws IOException
{
PrettyPrinter pp = new DefaultPrettyPrinter();
String LF = System.getProperty("line.separator");
String EXP = "{" + LF +
" \"name\" : \"John Doe\"," + LF +
" \"age\" : 3.14" + LF +
"}";
assertEquals(EXP, _printTestData(pp, false));
assertEquals(EXP, _printTestData(pp, true));
}
public void testWithLineFeed() throws IOException
{
PrettyPrinter pp = new DefaultPrettyPrinter()
.withObjectIndenter(new DefaultIndenter().withLinefeed("\n"));
String EXP = "{\n" +
" \"name\" : \"John Doe\",\n" +
" \"age\" : 3.14\n" +
"}";
assertEquals(EXP, _printTestData(pp, false));
assertEquals(EXP, _printTestData(pp, true));
}
public void testWithIndent() throws IOException
{
PrettyPrinter pp = new DefaultPrettyPrinter()
.withObjectIndenter(new DefaultIndenter().withLinefeed("\n").withIndent(" "));
String EXP = "{\n" +
" \"name\" : \"John Doe\",\n" +
" \"age\" : 3.14\n" +
"}";
assertEquals(EXP, _printTestData(pp, false));
assertEquals(EXP, _printTestData(pp, true));
}
public void testUnixLinefeed() throws IOException
{
PrettyPrinter pp = new DefaultPrettyPrinter()
.withObjectIndenter(new DefaultIndenter(" ", "\n"));
String EXP = "{\n" +
" \"name\" : \"John Doe\",\n" +
" \"age\" : 3.14\n" +
"}";
assertEquals(EXP, _printTestData(pp, false));
assertEquals(EXP, _printTestData(pp, true));
}
public void testWindowsLinefeed() throws IOException
{
PrettyPrinter pp = new DefaultPrettyPrinter()
.withObjectIndenter(new DefaultIndenter(" ", "\r\n"));
String EXP = "{\r\n" +
" \"name\" : \"John Doe\",\r\n" +
" \"age\" : 3.14\r\n" +
"}";
assertEquals(EXP, _printTestData(pp, false));
assertEquals(EXP, _printTestData(pp, true));
}
public void testTabIndent() throws IOException
{
PrettyPrinter pp = new DefaultPrettyPrinter()
.withObjectIndenter(new DefaultIndenter("\t", "\n"));
String EXP = "{\n" +
"\t\"name\" : \"John Doe\",\n" +
"\t\"age\" : 3.14\n" +
"}";
assertEquals(EXP, _printTestData(pp, false));
assertEquals(EXP, _printTestData(pp, true));
}
public void testRootSeparator() throws IOException
{
DefaultPrettyPrinter pp = new DefaultPrettyPrinter()
.withRootSeparator("|");
final String EXP = "1|2|3";
StringWriter sw = new StringWriter();
JsonGenerator gen = JSON_F.createGenerator(sw);
gen.setPrettyPrinter(pp);
gen.writeNumber(1);
gen.writeNumber(2);
gen.writeNumber(3);
gen.close();
assertEquals(EXP, sw.toString());
ByteArrayOutputStream bytes = new ByteArrayOutputStream();
gen = JSON_F.createGenerator(bytes);
gen.setPrettyPrinter(pp);
gen.writeNumber(1);
gen.writeNumber(2);
gen.writeNumber(3);
gen.close();
assertEquals(EXP, bytes.toString("UTF-8"));
// Also: let's try removing separator altogether
pp = pp.withRootSeparator((String) null)
.withArrayIndenter(null)
.withObjectIndenter(null)
.withoutSpacesInObjectEntries();
sw = new StringWriter();
gen = JSON_F.createGenerator(sw);
gen.setPrettyPrinter(pp);
gen.writeNumber(1);
gen.writeStartArray();
gen.writeNumber(2);
gen.writeEndArray();
gen.writeStartObject();
gen.writeFieldName("a");
gen.writeNumber(3);
gen.writeEndObject();
gen.close();
// no root separator, nor array, object
assertEquals("1[2]{\"a\":3}", sw.toString());
}
private String _printTestData(PrettyPrinter pp, boolean useBytes) throws IOException
{
JsonGenerator gen;
StringWriter sw;
ByteArrayOutputStream bytes;
if (useBytes) {
sw = null;
bytes = new ByteArrayOutputStream();
gen = JSON_F.createGenerator(bytes);
} else {
sw = new StringWriter();
bytes = null;
gen = JSON_F.createGenerator(sw);
}
gen.setPrettyPrinter(pp);
gen.writeStartObject();
gen.writeFieldName("name");
gen.writeString("John Doe");
gen.writeFieldName("age");
gen.writeNumber(3.14);
gen.writeEndObject();
gen.close();
if (useBytes) {
return bytes.toString("UTF-8");
}
return sw.toString();
}
// [core#502]: Force sub-classes to reimplement `createInstance`
public void testInvalidSubClass() throws Exception
{
DefaultPrettyPrinter pp = new MyPrettyPrinter();
try {
pp.createInstance();
fail("Should not pass");
} catch (IllegalStateException e) {
verifyException(e, "does not override");
}
}
@SuppressWarnings("serial")
static class MyPrettyPrinter extends DefaultPrettyPrinter { }
} |
|
public void testPrintInOperatorInForLoop() {
// Check for in expression in for's init expression.
// Check alone, with + (higher precedence), with ?: (lower precedence),
// and with conditional.
assertPrint("var a={}; for (var i = (\"length\" in a); i;) {}",
"var a={};for(var i=(\"length\"in a);i;);");
assertPrint("var a={}; for (var i = (\"length\" in a) ? 0 : 1; i;) {}",
"var a={};for(var i=(\"length\"in a)?0:1;i;);");
assertPrint("var a={}; for (var i = (\"length\" in a) + 1; i;) {}",
"var a={};for(var i=(\"length\"in a)+1;i;);");
assertPrint("var a={};for (var i = (\"length\" in a|| \"size\" in a);;);",
"var a={};for(var i=(\"length\"in a)||(\"size\"in a);;);");
assertPrint("var a={};for (var i = a || a || (\"size\" in a);;);",
"var a={};for(var i=a||a||(\"size\"in a);;);");
// Test works with unary operators and calls.
assertPrint("var a={}; for (var i = -(\"length\" in a); i;) {}",
"var a={};for(var i=-(\"length\"in a);i;);");
assertPrint("var a={};function b_(p){ return p;};" +
"for(var i=1,j=b_(\"length\" in a);;) {}",
"var a={};function b_(p){return p}" +
"for(var i=1,j=b_(\"length\"in a);;);");
// Test we correctly handle an in operator in the test clause.
assertPrint("var a={}; for (;(\"length\" in a);) {}",
"var a={};for(;\"length\"in a;);");
// Test we correctly handle an in operator inside a comma.
assertPrintSame("for(x,(y in z);;)foo()");
assertPrintSame("for(var x,w=(y in z);;)foo()");
// And in operator inside a hook.
assertPrintSame("for(a=c?0:(0 in d);;)foo()");
} | com.google.javascript.jscomp.CodePrinterTest::testPrintInOperatorInForLoop | test/com/google/javascript/jscomp/CodePrinterTest.java | 471 | test/com/google/javascript/jscomp/CodePrinterTest.java | testPrintInOperatorInForLoop | /*
* Copyright 2004 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import com.google.common.base.Joiner;
import com.google.common.collect.Lists;
import com.google.javascript.jscomp.CompilerOptions.LanguageMode;
import com.google.javascript.rhino.InputId;
import com.google.javascript.rhino.Node;
import com.google.javascript.rhino.Token;
import junit.framework.TestCase;
import java.util.List;
public class CodePrinterTest extends TestCase {
private boolean trustedStrings = true;
private Compiler lastCompiler = null;
private LanguageMode languageMode = LanguageMode.ECMASCRIPT5;
@Override public void setUp() {
trustedStrings = true;
lastCompiler = null;
languageMode = LanguageMode.ECMASCRIPT5;
}
Node parse(String js) {
return parse(js, false);
}
Node parse(String js, boolean checkTypes) {
Compiler compiler = new Compiler();
lastCompiler = compiler;
CompilerOptions options = new CompilerOptions();
options.setTrustedStrings(trustedStrings);
// Allow getters and setters.
options.setLanguageIn(LanguageMode.ECMASCRIPT5);
compiler.initOptions(options);
Node n = compiler.parseTestCode(js);
if (checkTypes) {
DefaultPassConfig passConfig = new DefaultPassConfig(null);
CompilerPass typeResolver = passConfig.resolveTypes.create(compiler);
Node externs = new Node(Token.SCRIPT);
externs.setInputId(new InputId("externs"));
Node externAndJsRoot = new Node(Token.BLOCK, externs, n);
externAndJsRoot.setIsSyntheticBlock(true);
typeResolver.process(externs, n);
CompilerPass inferTypes = passConfig.inferTypes.create(compiler);
inferTypes.process(externs, n);
}
checkUnexpectedErrorsOrWarnings(compiler, 0);
return n;
}
private static void checkUnexpectedErrorsOrWarnings(
Compiler compiler, int expected) {
int actual = compiler.getErrors().length + compiler.getWarnings().length;
if (actual != expected) {
String msg = "";
for (JSError err : compiler.getErrors()) {
msg += "Error:" + err.toString() + "\n";
}
for (JSError err : compiler.getWarnings()) {
msg += "Warning:" + err.toString() + "\n";
}
assertEquals("Unexpected warnings or errors.\n " + msg, expected, actual);
}
}
String parsePrint(String js, boolean prettyprint, int lineThreshold) {
CompilerOptions options = new CompilerOptions();
options.setTrustedStrings(trustedStrings);
options.setPrettyPrint(prettyprint);
options.setLineLengthThreshold(lineThreshold);
options.setLanguageOut(languageMode);
return new CodePrinter.Builder(parse(js)).setCompilerOptions(options)
.build();
}
String parsePrint(String js, boolean prettyprint, boolean lineBreak,
int lineThreshold) {
CompilerOptions options = new CompilerOptions();
options.setTrustedStrings(trustedStrings);
options.setPrettyPrint(prettyprint);
options.setLineLengthThreshold(lineThreshold);
options.setLineBreak(lineBreak);
options.setLanguageOut(languageMode);
return new CodePrinter.Builder(parse(js)).setCompilerOptions(options)
.build();
}
String parsePrint(String js, boolean prettyprint, boolean lineBreak,
boolean preferLineBreakAtEof, int lineThreshold) {
CompilerOptions options = new CompilerOptions();
options.setTrustedStrings(trustedStrings);
options.setPrettyPrint(prettyprint);
options.setLineLengthThreshold(lineThreshold);
options.setPreferLineBreakAtEndOfFile(preferLineBreakAtEof);
options.setLineBreak(lineBreak);
options.setLanguageOut(languageMode);
return new CodePrinter.Builder(parse(js)).setCompilerOptions(options)
.build();
}
String parsePrint(String js, boolean prettyprint, boolean lineBreak,
int lineThreshold, boolean outputTypes) {
Node node = parse(js, true);
CompilerOptions options = new CompilerOptions();
options.setTrustedStrings(trustedStrings);
options.setPrettyPrint(prettyprint);
options.setLineLengthThreshold(lineThreshold);
options.setLineBreak(lineBreak);
options.setLanguageOut(languageMode);
return new CodePrinter.Builder(node).setCompilerOptions(options)
.setOutputTypes(outputTypes)
.setTypeRegistry(lastCompiler.getTypeRegistry())
.build();
}
String parsePrint(String js, boolean prettyprint, boolean lineBreak,
int lineThreshold, boolean outputTypes,
boolean tagAsStrict) {
Node node = parse(js, true);
CompilerOptions options = new CompilerOptions();
options.setTrustedStrings(trustedStrings);
options.setPrettyPrint(prettyprint);
options.setLineLengthThreshold(lineThreshold);
options.setLineBreak(lineBreak);
options.setLanguageOut(languageMode);
return new CodePrinter.Builder(node).setCompilerOptions(options)
.setOutputTypes(outputTypes)
.setTypeRegistry(lastCompiler.getTypeRegistry())
.setTagAsStrict(tagAsStrict)
.build();
}
String printNode(Node n) {
CompilerOptions options = new CompilerOptions();
options.setLineLengthThreshold(CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD);
options.setLanguageOut(languageMode);
return new CodePrinter.Builder(n).setCompilerOptions(options).build();
}
void assertPrintNode(String expectedJs, Node ast) {
assertEquals(expectedJs, printNode(ast));
}
public void testPrint() {
assertPrint("10 + a + b", "10+a+b");
assertPrint("10 + (30*50)", "10+30*50");
assertPrint("with(x) { x + 3; }", "with(x)x+3");
assertPrint("\"aa'a\"", "\"aa'a\"");
assertPrint("\"aa\\\"a\"", "'aa\"a'");
assertPrint("function foo()\n{return 10;}", "function foo(){return 10}");
assertPrint("a instanceof b", "a instanceof b");
assertPrint("typeof(a)", "typeof a");
assertPrint(
"var foo = x ? { a : 1 } : {a: 3, b:4, \"default\": 5, \"foo-bar\": 6}",
"var foo=x?{a:1}:{a:3,b:4,\"default\":5,\"foo-bar\":6}");
// Safari: needs ';' at the end of a throw statement
assertPrint("function foo(){throw 'error';}",
"function foo(){throw\"error\";}");
// Safari 3 needs a "{" around a single function
assertPrint("if (true) function foo(){return}",
"if(true){function foo(){return}}");
assertPrint("var x = 10; { var y = 20; }", "var x=10;var y=20");
assertPrint("while (x-- > 0);", "while(x-- >0);");
assertPrint("x-- >> 1", "x-- >>1");
assertPrint("(function () {})(); ",
"(function(){})()");
// Associativity
assertPrint("var a,b,c,d;a || (b&& c) && (a || d)",
"var a,b,c,d;a||b&&c&&(a||d)");
assertPrint("var a,b,c; a || (b || c); a * (b * c); a | (b | c)",
"var a,b,c;a||b||c;a*b*c;a|b|c");
assertPrint("var a,b,c; a / b / c;a / (b / c); a - (b - c);",
"var a,b,c;a/b/c;a/(b/c);a-(b-c)");
assertPrint("var a,b; a = b = 3;",
"var a,b;a=b=3");
assertPrint("var a,b,c,d; a = (b = c = (d = 3));",
"var a,b,c,d;a=b=c=d=3");
assertPrint("var a,b,c; a += (b = c += 3);",
"var a,b,c;a+=b=c+=3");
assertPrint("var a,b,c; a *= (b -= c);",
"var a,b,c;a*=b-=c");
// Precedence
assertPrint("a ? delete b[0] : 3", "a?delete b[0]:3");
assertPrint("(delete a[0])/10", "delete a[0]/10");
// optional '()' for new
// simple new
assertPrint("new A", "new A");
assertPrint("new A()", "new A");
assertPrint("new A('x')", "new A(\"x\")");
// calling instance method directly after new
assertPrint("new A().a()", "(new A).a()");
assertPrint("(new A).a()", "(new A).a()");
// this case should be fixed
assertPrint("new A('y').a()", "(new A(\"y\")).a()");
// internal class
assertPrint("new A.B", "new A.B");
assertPrint("new A.B()", "new A.B");
assertPrint("new A.B('z')", "new A.B(\"z\")");
// calling instance method directly after new internal class
assertPrint("(new A.B).a()", "(new A.B).a()");
assertPrint("new A.B().a()", "(new A.B).a()");
// this case should be fixed
assertPrint("new A.B('w').a()", "(new A.B(\"w\")).a()");
// Operators: make sure we don't convert binary + and unary + into ++
assertPrint("x + +y", "x+ +y");
assertPrint("x - (-y)", "x- -y");
assertPrint("x++ +y", "x++ +y");
assertPrint("x-- -y", "x-- -y");
assertPrint("x++ -y", "x++-y");
// Label
assertPrint("foo:for(;;){break foo;}", "foo:for(;;)break foo");
assertPrint("foo:while(1){continue foo;}", "foo:while(1)continue foo");
// Object literals.
assertPrint("({})", "({})");
assertPrint("var x = {};", "var x={}");
assertPrint("({}).x", "({}).x");
assertPrint("({})['x']", "({})[\"x\"]");
assertPrint("({}) instanceof Object", "({})instanceof Object");
assertPrint("({}) || 1", "({})||1");
assertPrint("1 || ({})", "1||{}");
assertPrint("({}) ? 1 : 2", "({})?1:2");
assertPrint("0 ? ({}) : 2", "0?{}:2");
assertPrint("0 ? 1 : ({})", "0?1:{}");
assertPrint("typeof ({})", "typeof{}");
assertPrint("f({})", "f({})");
// Anonymous function expressions.
assertPrint("(function(){})", "(function(){})");
assertPrint("(function(){})()", "(function(){})()");
assertPrint("(function(){})instanceof Object",
"(function(){})instanceof Object");
assertPrint("(function(){}).bind().call()",
"(function(){}).bind().call()");
assertPrint("var x = function() { };", "var x=function(){}");
assertPrint("var x = function() { }();", "var x=function(){}()");
assertPrint("(function() {}), 2", "(function(){}),2");
// Name functions expression.
assertPrint("(function f(){})", "(function f(){})");
// Function declaration.
assertPrint("function f(){}", "function f(){}");
// Make sure we don't treat non-Latin character escapes as raw strings.
assertPrint("({ 'a': 4, '\\u0100': 4 })", "({\"a\":4,\"\\u0100\":4})");
assertPrint("({ a: 4, '\\u0100': 4 })", "({a:4,\"\\u0100\":4})");
// Test if statement and for statements with single statements in body.
assertPrint("if (true) { alert();}", "if(true)alert()");
assertPrint("if (false) {} else {alert(\"a\");}",
"if(false);else alert(\"a\")");
assertPrint("for(;;) { alert();};", "for(;;)alert()");
assertPrint("do { alert(); } while(true);",
"do alert();while(true)");
assertPrint("myLabel: { alert();}",
"myLabel:alert()");
assertPrint("myLabel: for(;;) continue myLabel;",
"myLabel:for(;;)continue myLabel");
// Test nested var statement
assertPrint("if (true) var x; x = 4;", "if(true)var x;x=4");
// Non-latin identifier. Make sure we keep them escaped.
assertPrint("\\u00fb", "\\u00fb");
assertPrint("\\u00fa=1", "\\u00fa=1");
assertPrint("function \\u00f9(){}", "function \\u00f9(){}");
assertPrint("x.\\u00f8", "x.\\u00f8");
assertPrint("x.\\u00f8", "x.\\u00f8");
assertPrint("abc\\u4e00\\u4e01jkl", "abc\\u4e00\\u4e01jkl");
// Test the right-associative unary operators for spurious parens
assertPrint("! ! true", "!!true");
assertPrint("!(!(true))", "!!true");
assertPrint("typeof(void(0))", "typeof void 0");
assertPrint("typeof(void(!0))", "typeof void!0");
assertPrint("+ - + + - + 3", "+-+ +-+3"); // chained unary plus/minus
assertPrint("+(--x)", "+--x");
assertPrint("-(++x)", "-++x");
// needs a space to prevent an ambiguous parse
assertPrint("-(--x)", "- --x");
assertPrint("!(~~5)", "!~~5");
assertPrint("~(a/b)", "~(a/b)");
// Preserve parens to overcome greedy binding of NEW
assertPrint("new (foo.bar()).factory(baz)", "new (foo.bar().factory)(baz)");
assertPrint("new (bar()).factory(baz)", "new (bar().factory)(baz)");
assertPrint("new (new foobar(x)).factory(baz)",
"new (new foobar(x)).factory(baz)");
// Make sure that HOOK is right associative
assertPrint("a ? b : (c ? d : e)", "a?b:c?d:e");
assertPrint("a ? (b ? c : d) : e", "a?b?c:d:e");
assertPrint("(a ? b : c) ? d : e", "(a?b:c)?d:e");
// Test nested ifs
assertPrint("if (x) if (y); else;", "if(x)if(y);else;");
// Test comma.
assertPrint("a,b,c", "a,b,c");
assertPrint("(a,b),c", "a,b,c");
assertPrint("a,(b,c)", "a,b,c");
assertPrint("x=a,b,c", "x=a,b,c");
assertPrint("x=(a,b),c", "x=(a,b),c");
assertPrint("x=a,(b,c)", "x=a,b,c");
assertPrint("x=a,y=b,z=c", "x=a,y=b,z=c");
assertPrint("x=(a,y=b,z=c)", "x=(a,y=b,z=c)");
assertPrint("x=[a,b,c,d]", "x=[a,b,c,d]");
assertPrint("x=[(a,b,c),d]", "x=[(a,b,c),d]");
assertPrint("x=[(a,(b,c)),d]", "x=[(a,b,c),d]");
assertPrint("x=[a,(b,c,d)]", "x=[a,(b,c,d)]");
assertPrint("var x=(a,b)", "var x=(a,b)");
assertPrint("var x=a,b,c", "var x=a,b,c");
assertPrint("var x=(a,b),c", "var x=(a,b),c");
assertPrint("var x=a,b=(c,d)", "var x=a,b=(c,d)");
assertPrint("foo(a,b,c,d)", "foo(a,b,c,d)");
assertPrint("foo((a,b,c),d)", "foo((a,b,c),d)");
assertPrint("foo((a,(b,c)),d)", "foo((a,b,c),d)");
assertPrint("f(a+b,(c,d,(e,f,g)))", "f(a+b,(c,d,e,f,g))");
assertPrint("({}) , 1 , 2", "({}),1,2");
assertPrint("({}) , {} , {}", "({}),{},{}");
// EMPTY nodes
assertPrint("if (x){}", "if(x);");
assertPrint("if(x);", "if(x);");
assertPrint("if(x)if(y);", "if(x)if(y);");
assertPrint("if(x){if(y);}", "if(x)if(y);");
assertPrint("if(x){if(y){};;;}", "if(x)if(y);");
assertPrint("if(x){;;function y(){};;}", "if(x){function y(){}}");
}
public void testBreakTrustedStrings() {
// Break scripts
assertPrint("'<script>'", "\"<script>\"");
assertPrint("'</script>'", "\"\\x3c/script>\"");
assertPrint("\"</script> </SCRIPT>\"", "\"\\x3c/script> \\x3c/SCRIPT>\"");
assertPrint("'-->'", "\"--\\x3e\"");
assertPrint("']]>'", "\"]]\\x3e\"");
assertPrint("' --></script>'", "\" --\\x3e\\x3c/script>\"");
assertPrint("/--> <\\/script>/g", "/--\\x3e <\\/script>/g");
// Break HTML start comments. Certain versions of WebKit
// begin an HTML comment when they see this.
assertPrint("'<!-- I am a string -->'",
"\"\\x3c!-- I am a string --\\x3e\"");
assertPrint("'<=&>'", "\"<=&>\"");
}
public void testBreakUntrustedStrings() {
trustedStrings = false;
// Break scripts
assertPrint("'<script>'", "\"\\x3cscript\\x3e\"");
assertPrint("'</script>'", "\"\\x3c/script\\x3e\"");
assertPrint("\"</script> </SCRIPT>\"", "\"\\x3c/script\\x3e \\x3c/SCRIPT\\x3e\"");
assertPrint("'-->'", "\"--\\x3e\"");
assertPrint("']]>'", "\"]]\\x3e\"");
assertPrint("' --></script>'", "\" --\\x3e\\x3c/script\\x3e\"");
assertPrint("/--> <\\/script>/g", "/--\\x3e <\\/script>/g");
// Break HTML start comments. Certain versions of WebKit
// begin an HTML comment when they see this.
assertPrint("'<!-- I am a string -->'",
"\"\\x3c!-- I am a string --\\x3e\"");
assertPrint("'<=&>'", "\"\\x3c\\x3d\\x26\\x3e\"");
assertPrint("/(?=x)/", "/(?=x)/");
}
public void testPrintArray() {
assertPrint("[void 0, void 0]", "[void 0,void 0]");
assertPrint("[undefined, undefined]", "[undefined,undefined]");
assertPrint("[ , , , undefined]", "[,,,undefined]");
assertPrint("[ , , , 0]", "[,,,0]");
}
public void testHook() {
assertPrint("a ? b = 1 : c = 2", "a?b=1:c=2");
assertPrint("x = a ? b = 1 : c = 2", "x=a?b=1:c=2");
assertPrint("(x = a) ? b = 1 : c = 2", "(x=a)?b=1:c=2");
assertPrint("x, a ? b = 1 : c = 2", "x,a?b=1:c=2");
assertPrint("x, (a ? b = 1 : c = 2)", "x,a?b=1:c=2");
assertPrint("(x, a) ? b = 1 : c = 2", "(x,a)?b=1:c=2");
assertPrint("a ? (x, b) : c = 2", "a?(x,b):c=2");
assertPrint("a ? b = 1 : (x,c)", "a?b=1:(x,c)");
assertPrint("a ? b = 1 : c = 2 + x", "a?b=1:c=2+x");
assertPrint("(a ? b = 1 : c = 2) + x", "(a?b=1:c=2)+x");
assertPrint("a ? b = 1 : (c = 2) + x", "a?b=1:(c=2)+x");
assertPrint("a ? (b?1:2) : 3", "a?b?1:2:3");
}
public void testPrintInOperatorInForLoop() {
// Check for in expression in for's init expression.
// Check alone, with + (higher precedence), with ?: (lower precedence),
// and with conditional.
assertPrint("var a={}; for (var i = (\"length\" in a); i;) {}",
"var a={};for(var i=(\"length\"in a);i;);");
assertPrint("var a={}; for (var i = (\"length\" in a) ? 0 : 1; i;) {}",
"var a={};for(var i=(\"length\"in a)?0:1;i;);");
assertPrint("var a={}; for (var i = (\"length\" in a) + 1; i;) {}",
"var a={};for(var i=(\"length\"in a)+1;i;);");
assertPrint("var a={};for (var i = (\"length\" in a|| \"size\" in a);;);",
"var a={};for(var i=(\"length\"in a)||(\"size\"in a);;);");
assertPrint("var a={};for (var i = a || a || (\"size\" in a);;);",
"var a={};for(var i=a||a||(\"size\"in a);;);");
// Test works with unary operators and calls.
assertPrint("var a={}; for (var i = -(\"length\" in a); i;) {}",
"var a={};for(var i=-(\"length\"in a);i;);");
assertPrint("var a={};function b_(p){ return p;};" +
"for(var i=1,j=b_(\"length\" in a);;) {}",
"var a={};function b_(p){return p}" +
"for(var i=1,j=b_(\"length\"in a);;);");
// Test we correctly handle an in operator in the test clause.
assertPrint("var a={}; for (;(\"length\" in a);) {}",
"var a={};for(;\"length\"in a;);");
// Test we correctly handle an in operator inside a comma.
assertPrintSame("for(x,(y in z);;)foo()");
assertPrintSame("for(var x,w=(y in z);;)foo()");
// And in operator inside a hook.
assertPrintSame("for(a=c?0:(0 in d);;)foo()");
}
public void testLiteralProperty() {
assertPrint("(64).toString()", "(64).toString()");
}
private void assertPrint(String js, String expected) {
parse(expected); // validate the expected string is valid JS
assertEquals(expected,
parsePrint(js, false, CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD));
}
private void assertPrintSame(String js) {
assertPrint(js, js);
}
// Make sure that the code generator doesn't associate an
// else clause with the wrong if clause.
public void testAmbiguousElseClauses() {
assertPrintNode("if(x)if(y);else;",
new Node(Token.IF,
Node.newString(Token.NAME, "x"),
new Node(Token.BLOCK,
new Node(Token.IF,
Node.newString(Token.NAME, "y"),
new Node(Token.BLOCK),
// ELSE clause for the inner if
new Node(Token.BLOCK)))));
assertPrintNode("if(x){if(y);}else;",
new Node(Token.IF,
Node.newString(Token.NAME, "x"),
new Node(Token.BLOCK,
new Node(Token.IF,
Node.newString(Token.NAME, "y"),
new Node(Token.BLOCK))),
// ELSE clause for the outer if
new Node(Token.BLOCK)));
assertPrintNode("if(x)if(y);else{if(z);}else;",
new Node(Token.IF,
Node.newString(Token.NAME, "x"),
new Node(Token.BLOCK,
new Node(Token.IF,
Node.newString(Token.NAME, "y"),
new Node(Token.BLOCK),
new Node(Token.BLOCK,
new Node(Token.IF,
Node.newString(Token.NAME, "z"),
new Node(Token.BLOCK))))),
// ELSE clause for the outermost if
new Node(Token.BLOCK)));
}
public void testLineBreak() {
// line break after function if in a statement context
assertLineBreak("function a() {}\n" +
"function b() {}",
"function a(){}\n" +
"function b(){}\n");
// line break after ; after a function
assertLineBreak("var a = {};\n" +
"a.foo = function () {}\n" +
"function b() {}",
"var a={};a.foo=function(){};\n" +
"function b(){}\n");
// break after comma after a function
assertLineBreak("var a = {\n" +
" b: function() {},\n" +
" c: function() {}\n" +
"};\n" +
"alert(a);",
"var a={b:function(){},\n" +
"c:function(){}};\n" +
"alert(a)");
}
private void assertLineBreak(String js, String expected) {
assertEquals(expected,
parsePrint(js, false, true,
CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD));
}
public void testPreferLineBreakAtEndOfFile() {
// short final line, no previous break, do nothing
assertLineBreakAtEndOfFile(
"\"1234567890\";",
"\"1234567890\"",
"\"1234567890\"");
// short final line, shift previous break to end
assertLineBreakAtEndOfFile(
"\"123456789012345678901234567890\";\"1234567890\"",
"\"123456789012345678901234567890\";\n\"1234567890\"",
"\"123456789012345678901234567890\"; \"1234567890\";\n");
assertLineBreakAtEndOfFile(
"var12345678901234567890123456 instanceof Object;",
"var12345678901234567890123456 instanceof\nObject",
"var12345678901234567890123456 instanceof Object;\n");
// long final line, no previous break, add a break at end
assertLineBreakAtEndOfFile(
"\"1234567890\";\"12345678901234567890\";",
"\"1234567890\";\"12345678901234567890\"",
"\"1234567890\";\"12345678901234567890\";\n");
// long final line, previous break, add a break at end
assertLineBreakAtEndOfFile(
"\"123456789012345678901234567890\";\"12345678901234567890\";",
"\"123456789012345678901234567890\";\n\"12345678901234567890\"",
"\"123456789012345678901234567890\";\n\"12345678901234567890\";\n");
}
private void assertLineBreakAtEndOfFile(String js,
String expectedWithoutBreakAtEnd, String expectedWithBreakAtEnd) {
assertEquals(expectedWithoutBreakAtEnd,
parsePrint(js, false, false, false, 30));
assertEquals(expectedWithBreakAtEnd,
parsePrint(js, false, false, true, 30));
}
public void testPrettyPrinter() {
// Ensure that the pretty printer inserts line breaks at appropriate
// places.
assertPrettyPrint("(function(){})();","(function() {\n})();\n");
assertPrettyPrint("var a = (function() {});alert(a);",
"var a = function() {\n};\nalert(a);\n");
// Check we correctly handle putting brackets around all if clauses so
// we can put breakpoints inside statements.
assertPrettyPrint("if (1) {}",
"if(1) {\n" +
"}\n");
assertPrettyPrint("if (1) {alert(\"\");}",
"if(1) {\n" +
" alert(\"\")\n" +
"}\n");
assertPrettyPrint("if (1)alert(\"\");",
"if(1) {\n" +
" alert(\"\")\n" +
"}\n");
assertPrettyPrint("if (1) {alert();alert();}",
"if(1) {\n" +
" alert();\n" +
" alert()\n" +
"}\n");
// Don't add blocks if they weren't there already.
assertPrettyPrint("label: alert();",
"label:alert();\n");
// But if statements and loops get blocks automagically.
assertPrettyPrint("if (1) alert();",
"if(1) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint("for (;;) alert();",
"for(;;) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint("while (1) alert();",
"while(1) {\n" +
" alert()\n" +
"}\n");
// Do we put else clauses in blocks?
assertPrettyPrint("if (1) {} else {alert(a);}",
"if(1) {\n" +
"}else {\n alert(a)\n}\n");
// Do we add blocks to else clauses?
assertPrettyPrint("if (1) alert(a); else alert(b);",
"if(1) {\n" +
" alert(a)\n" +
"}else {\n" +
" alert(b)\n" +
"}\n");
// Do we put for bodies in blocks?
assertPrettyPrint("for(;;) { alert();}",
"for(;;) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint("for(;;) {}",
"for(;;) {\n" +
"}\n");
assertPrettyPrint("for(;;) { alert(); alert(); }",
"for(;;) {\n" +
" alert();\n" +
" alert()\n" +
"}\n");
// How about do loops?
assertPrettyPrint("do { alert(); } while(true);",
"do {\n" +
" alert()\n" +
"}while(true);\n");
// label?
assertPrettyPrint("myLabel: { alert();}",
"myLabel: {\n" +
" alert()\n" +
"}\n");
// Don't move the label on a loop, because then break {label} and
// continue {label} won't work.
assertPrettyPrint("myLabel: for(;;) continue myLabel;",
"myLabel:for(;;) {\n" +
" continue myLabel\n" +
"}\n");
assertPrettyPrint("var a;", "var a;\n");
}
public void testPrettyPrinter2() {
assertPrettyPrint(
"if(true) f();",
"if(true) {\n" +
" f()\n" +
"}\n");
assertPrettyPrint(
"if (true) { f() } else { g() }",
"if(true) {\n" +
" f()\n" +
"}else {\n" +
" g()\n" +
"}\n");
assertPrettyPrint(
"if(true) f(); for(;;) g();",
"if(true) {\n" +
" f()\n" +
"}\n" +
"for(;;) {\n" +
" g()\n" +
"}\n");
}
public void testPrettyPrinter3() {
assertPrettyPrint(
"try {} catch(e) {}if (1) {alert();alert();}",
"try {\n" +
"}catch(e) {\n" +
"}\n" +
"if(1) {\n" +
" alert();\n" +
" alert()\n" +
"}\n");
assertPrettyPrint(
"try {} finally {}if (1) {alert();alert();}",
"try {\n" +
"}finally {\n" +
"}\n" +
"if(1) {\n" +
" alert();\n" +
" alert()\n" +
"}\n");
assertPrettyPrint(
"try {} catch(e) {} finally {} if (1) {alert();alert();}",
"try {\n" +
"}catch(e) {\n" +
"}finally {\n" +
"}\n" +
"if(1) {\n" +
" alert();\n" +
" alert()\n" +
"}\n");
}
public void testPrettyPrinter4() {
assertPrettyPrint(
"function f() {}if (1) {alert();}",
"function f() {\n" +
"}\n" +
"if(1) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint(
"var f = function() {};if (1) {alert();}",
"var f = function() {\n" +
"};\n" +
"if(1) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint(
"(function() {})();if (1) {alert();}",
"(function() {\n" +
"})();\n" +
"if(1) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint(
"(function() {alert();alert();})();if (1) {alert();}",
"(function() {\n" +
" alert();\n" +
" alert()\n" +
"})();\n" +
"if(1) {\n" +
" alert()\n" +
"}\n");
}
public void testTypeAnnotations() {
assertTypeAnnotations(
"/** @constructor */ function Foo(){}",
"/**\n * @return {undefined}\n * @constructor\n */\n"
+ "function Foo() {\n}\n");
}
public void testTypeAnnotationsTypeDef() {
// TODO(johnlenz): It would be nice if there were some way to preserve
// typedefs but currently they are resolved into the basic types in the
// type registry.
assertTypeAnnotations(
"/** @typedef {Array.<number>} */ goog.java.Long;\n"
+ "/** @param {!goog.java.Long} a*/\n"
+ "function f(a){};\n",
"goog.java.Long;\n"
+ "/**\n"
+ " * @param {(Array.<number>|null)} a\n"
+ " * @return {undefined}\n"
+ " */\n"
+ "function f(a) {\n}\n");
}
public void testTypeAnnotationsAssign() {
assertTypeAnnotations("/** @constructor */ var Foo = function(){}",
"/**\n * @return {undefined}\n * @constructor\n */\n"
+ "var Foo = function() {\n};\n");
}
public void testTypeAnnotationsNamespace() {
assertTypeAnnotations("var a = {};"
+ "/** @constructor */ a.Foo = function(){}",
"var a = {};\n"
+ "/**\n * @return {undefined}\n * @constructor\n */\n"
+ "a.Foo = function() {\n};\n");
}
public void testTypeAnnotationsMemberSubclass() {
assertTypeAnnotations("var a = {};"
+ "/** @constructor */ a.Foo = function(){};"
+ "/** @constructor \n @extends {a.Foo} */ a.Bar = function(){}",
"var a = {};\n"
+ "/**\n * @return {undefined}\n * @constructor\n */\n"
+ "a.Foo = function() {\n};\n"
+ "/**\n * @return {undefined}\n * @extends {a.Foo}\n"
+ " * @constructor\n */\n"
+ "a.Bar = function() {\n};\n");
}
public void testTypeAnnotationsInterface() {
assertTypeAnnotations("var a = {};"
+ "/** @interface */ a.Foo = function(){};"
+ "/** @interface \n @extends {a.Foo} */ a.Bar = function(){}",
"var a = {};\n"
+ "/**\n * @interface\n */\n"
+ "a.Foo = function() {\n};\n"
+ "/**\n * @extends {a.Foo}\n"
+ " * @interface\n */\n"
+ "a.Bar = function() {\n};\n");
}
public void testTypeAnnotationsMultipleInterface() {
assertTypeAnnotations("var a = {};"
+ "/** @interface */ a.Foo1 = function(){};"
+ "/** @interface */ a.Foo2 = function(){};"
+ "/** @interface \n @extends {a.Foo1} \n @extends {a.Foo2} */"
+ "a.Bar = function(){}",
"var a = {};\n"
+ "/**\n * @interface\n */\n"
+ "a.Foo1 = function() {\n};\n"
+ "/**\n * @interface\n */\n"
+ "a.Foo2 = function() {\n};\n"
+ "/**\n * @extends {a.Foo1}\n"
+ " * @extends {a.Foo2}\n"
+ " * @interface\n */\n"
+ "a.Bar = function() {\n};\n");
}
public void testTypeAnnotationsMember() {
assertTypeAnnotations("var a = {};"
+ "/** @constructor */ a.Foo = function(){}"
+ "/** @param {string} foo\n"
+ " * @return {number} */\n"
+ "a.Foo.prototype.foo = function(foo) { return 3; };"
+ "/** @type {string|undefined} */"
+ "a.Foo.prototype.bar = '';",
"var a = {};\n"
+ "/**\n * @return {undefined}\n * @constructor\n */\n"
+ "a.Foo = function() {\n};\n"
+ "/**\n"
+ " * @param {string} foo\n"
+ " * @return {number}\n"
+ " */\n"
+ "a.Foo.prototype.foo = function(foo) {\n return 3\n};\n"
+ "/** @type {string} */\n"
+ "a.Foo.prototype.bar = \"\";\n");
}
public void testTypeAnnotationsImplements() {
assertTypeAnnotations("var a = {};"
+ "/** @constructor */ a.Foo = function(){};\n"
+ "/** @interface */ a.I = function(){};\n"
+ "/** @interface */ a.I2 = function(){};\n"
+ "/** @constructor \n @extends {a.Foo}\n"
+ " * @implements {a.I} \n @implements {a.I2}\n"
+ "*/ a.Bar = function(){}",
"var a = {};\n"
+ "/**\n * @return {undefined}\n * @constructor\n */\n"
+ "a.Foo = function() {\n};\n"
+ "/**\n * @interface\n */\n"
+ "a.I = function() {\n};\n"
+ "/**\n * @interface\n */\n"
+ "a.I2 = function() {\n};\n"
+ "/**\n * @return {undefined}\n * @extends {a.Foo}\n"
+ " * @implements {a.I}\n"
+ " * @implements {a.I2}\n * @constructor\n */\n"
+ "a.Bar = function() {\n};\n");
}
public void testTypeAnnotationsDispatcher1() {
assertTypeAnnotations(
"var a = {};\n" +
"/** \n" +
" * @constructor \n" +
" * @javadispatch \n" +
" */\n" +
"a.Foo = function(){}",
"var a = {};\n" +
"/**\n" +
" * @return {undefined}\n" +
" * @constructor\n" +
" * @javadispatch\n" +
" */\n" +
"a.Foo = function() {\n" +
"};\n");
}
public void testTypeAnnotationsDispatcher2() {
assertTypeAnnotations(
"var a = {};\n" +
"/** \n" +
" * @constructor \n" +
" */\n" +
"a.Foo = function(){}\n" +
"/**\n" +
" * @javadispatch\n" +
" */\n" +
"a.Foo.prototype.foo = function() {};",
"var a = {};\n" +
"/**\n" +
" * @return {undefined}\n" +
" * @constructor\n" +
" */\n" +
"a.Foo = function() {\n" +
"};\n" +
"/**\n" +
" * @return {undefined}\n" +
" * @javadispatch\n" +
" */\n" +
"a.Foo.prototype.foo = function() {\n" +
"};\n");
}
public void testU2UFunctionTypeAnnotation1() {
assertTypeAnnotations(
"/** @type {!Function} */ var x = function() {}",
"/** @type {!Function} */\n" +
"var x = function() {\n};\n");
}
public void testU2UFunctionTypeAnnotation2() {
// TODO(johnlenz): we currently report the type of the RHS which is not
// correct, we should export the type of the LHS.
assertTypeAnnotations(
"/** @type {Function} */ var x = function() {}",
"/** @type {!Function} */\n" +
"var x = function() {\n};\n");
}
public void testEmitUnknownParamTypesAsAllType() {
assertTypeAnnotations(
"var a = function(x) {}",
"/**\n" +
" * @param {?} x\n" +
" * @return {undefined}\n" +
" */\n" +
"var a = function(x) {\n};\n");
}
public void testOptionalTypesAnnotation() {
assertTypeAnnotations(
"/**\n" +
" * @param {string=} x \n" +
" */\n" +
"var a = function(x) {}",
"/**\n" +
" * @param {string=} x\n" +
" * @return {undefined}\n" +
" */\n" +
"var a = function(x) {\n};\n");
}
public void testVariableArgumentsTypesAnnotation() {
assertTypeAnnotations(
"/**\n" +
" * @param {...string} x \n" +
" */\n" +
"var a = function(x) {}",
"/**\n" +
" * @param {...string} x\n" +
" * @return {undefined}\n" +
" */\n" +
"var a = function(x) {\n};\n");
}
public void testTempConstructor() {
assertTypeAnnotations(
"var x = function() {\n/**\n * @constructor\n */\nfunction t1() {}\n" +
" /**\n * @constructor\n */\nfunction t2() {}\n" +
" t1.prototype = t2.prototype}",
"/**\n * @return {undefined}\n */\nvar x = function() {\n" +
" /**\n * @return {undefined}\n * @constructor\n */\n" +
"function t1() {\n }\n" +
" /**\n * @return {undefined}\n * @constructor\n */\n" +
"function t2() {\n }\n" +
" t1.prototype = t2.prototype\n};\n"
);
}
public void testEnumAnnotation1() {
assertTypeAnnotations(
"/** @enum {string} */ var Enum = {FOO: 'x', BAR: 'y'};",
"/** @enum {string} */\nvar Enum = {FOO:\"x\", BAR:\"y\"};\n");
}
public void testEnumAnnotation2() {
assertTypeAnnotations(
"var goog = goog || {};" +
"/** @enum {string} */ goog.Enum = {FOO: 'x', BAR: 'y'};" +
"/** @const */ goog.Enum2 = goog.x ? {} : goog.Enum;",
"var goog = goog || {};\n" +
"/** @enum {string} */\ngoog.Enum = {FOO:\"x\", BAR:\"y\"};\n" +
"/** @type {(Object|{})} */\ngoog.Enum2 = goog.x ? {} : goog.Enum;\n");
}
private void assertPrettyPrint(String js, String expected) {
assertEquals(expected,
parsePrint(js, true, false,
CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD));
}
private void assertTypeAnnotations(String js, String expected) {
assertEquals(expected,
parsePrint(js, true, false,
CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD, true));
}
public void testSubtraction() {
Compiler compiler = new Compiler();
Node n = compiler.parseTestCode("x - -4");
assertEquals(0, compiler.getErrorCount());
assertEquals(
"x- -4",
printNode(n));
}
public void testFunctionWithCall() {
assertPrint(
"var user = new function() {"
+ "alert(\"foo\")}",
"var user=new function(){"
+ "alert(\"foo\")}");
assertPrint(
"var user = new function() {"
+ "this.name = \"foo\";"
+ "this.local = function(){alert(this.name)};}",
"var user=new function(){"
+ "this.name=\"foo\";"
+ "this.local=function(){alert(this.name)}}");
}
public void testLineLength() {
// list
assertLineLength("var aba,bcb,cdc",
"var aba,bcb," +
"\ncdc");
// operators, and two breaks
assertLineLength(
"\"foo\"+\"bar,baz,bomb\"+\"whee\"+\";long-string\"\n+\"aaa\"",
"\"foo\"+\"bar,baz,bomb\"+" +
"\n\"whee\"+\";long-string\"+" +
"\n\"aaa\"");
// assignment
assertLineLength("var abazaba=1234",
"var abazaba=" +
"\n1234");
// statements
assertLineLength("var abab=1;var bab=2",
"var abab=1;" +
"\nvar bab=2");
// don't break regexes
assertLineLength("var a=/some[reg](ex),with.*we?rd|chars/i;var b=a",
"var a=/some[reg](ex),with.*we?rd|chars/i;" +
"\nvar b=a");
// don't break strings
assertLineLength("var a=\"foo,{bar};baz\";var b=a",
"var a=\"foo,{bar};baz\";" +
"\nvar b=a");
// don't break before post inc/dec
assertLineLength("var a=\"a\";a++;var b=\"bbb\";",
"var a=\"a\";a++;\n" +
"var b=\"bbb\"");
}
private void assertLineLength(String js, String expected) {
assertEquals(expected,
parsePrint(js, false, true, 10));
}
public void testParsePrintParse() {
testReparse("3;");
testReparse("var a = b;");
testReparse("var x, y, z;");
testReparse("try { foo() } catch(e) { bar() }");
testReparse("try { foo() } catch(e) { bar() } finally { stuff() }");
testReparse("try { foo() } finally { stuff() }");
testReparse("throw 'me'");
testReparse("function foo(a) { return a + 4; }");
testReparse("function foo() { return; }");
testReparse("var a = function(a, b) { foo(); return a + b; }");
testReparse("b = [3, 4, 'paul', \"Buchhe it\",,5];");
testReparse("v = (5, 6, 7, 8)");
testReparse("d = 34.0; x = 0; y = .3; z = -22");
testReparse("d = -x; t = !x + ~y;");
testReparse("'hi'; /* just a test */ stuff(a,b) \n" +
" foo(); // and another \n" +
" bar();");
testReparse("a = b++ + ++c; a = b++-++c; a = - --b; a = - ++b;");
testReparse("a++; b= a++; b = ++a; b = a--; b = --a; a+=2; b-=5");
testReparse("a = (2 + 3) * 4;");
testReparse("a = 1 + (2 + 3) + 4;");
testReparse("x = a ? b : c; x = a ? (b,3,5) : (foo(),bar());");
testReparse("a = b | c || d ^ e " +
"&& f & !g != h << i <= j < k >>> l > m * n % !o");
testReparse("a == b; a != b; a === b; a == b == a;" +
" (a == b) == a; a == (b == a);");
testReparse("if (a > b) a = b; if (b < 3) a = 3; else c = 4;");
testReparse("if (a == b) { a++; } if (a == 0) { a++; } else { a --; }");
testReparse("for (var i in a) b += i;");
testReparse("for (var i = 0; i < 10; i++){ b /= 2;" +
" if (b == 2)break;else continue;}");
testReparse("for (x = 0; x < 10; x++) a /= 2;");
testReparse("for (;;) a++;");
testReparse("while(true) { blah(); }while(true) blah();");
testReparse("do stuff(); while(a>b);");
testReparse("[0, null, , true, false, this];");
testReparse("s.replace(/absc/, 'X').replace(/ab/gi, 'Y');");
testReparse("new Foo; new Bar(a, b,c);");
testReparse("with(foo()) { x = z; y = t; } with(bar()) a = z;");
testReparse("delete foo['bar']; delete foo;");
testReparse("var x = { 'a':'paul', 1:'3', 2:(3,4) };");
testReparse("switch(a) { case 2: case 3: stuff(); break;" +
"case 4: morestuff(); break; default: done();}");
testReparse("x = foo['bar'] + foo['my stuff'] + foo[bar] + f.stuff;");
testReparse("a.v = b.v; x['foo'] = y['zoo'];");
testReparse("'test' in x; 3 in x; a in x;");
testReparse("'foo\"bar' + \"foo'c\" + 'stuff\\n and \\\\more'");
testReparse("x.__proto__;");
}
private void testReparse(String code) {
Compiler compiler = new Compiler();
Node parse1 = parse(code);
Node parse2 = parse(new CodePrinter.Builder(parse1).build());
String explanation = parse1.checkTreeEquals(parse2);
assertNull("\nExpected: " + compiler.toSource(parse1) +
"\nResult: " + compiler.toSource(parse2) +
"\n" + explanation, explanation);
}
public void testDoLoopIECompatiblity() {
// Do loops within IFs cause syntax errors in IE6 and IE7.
assertPrint("function f(){if(e1){do foo();while(e2)}else foo()}",
"function f(){if(e1){do foo();while(e2)}else foo()}");
assertPrint("function f(){if(e1)do foo();while(e2)else foo()}",
"function f(){if(e1){do foo();while(e2)}else foo()}");
assertPrint("if(x){do{foo()}while(y)}else bar()",
"if(x){do foo();while(y)}else bar()");
assertPrint("if(x)do{foo()}while(y);else bar()",
"if(x){do foo();while(y)}else bar()");
assertPrint("if(x){do{foo()}while(y)}",
"if(x){do foo();while(y)}");
assertPrint("if(x)do{foo()}while(y);",
"if(x){do foo();while(y)}");
assertPrint("if(x)A:do{foo()}while(y);",
"if(x){A:do foo();while(y)}");
assertPrint("var i = 0;a: do{b: do{i++;break b;} while(0);} while(0);",
"var i=0;a:do{b:do{i++;break b}while(0)}while(0)");
}
public void testFunctionSafariCompatiblity() {
// Functions within IFs cause syntax errors on Safari.
assertPrint("function f(){if(e1){function goo(){return true}}else foo()}",
"function f(){if(e1){function goo(){return true}}else foo()}");
assertPrint("function f(){if(e1)function goo(){return true}else foo()}",
"function f(){if(e1){function goo(){return true}}else foo()}");
assertPrint("if(e1){function goo(){return true}}",
"if(e1){function goo(){return true}}");
assertPrint("if(e1)function goo(){return true}",
"if(e1){function goo(){return true}}");
assertPrint("if(e1)A:function goo(){return true}",
"if(e1){A:function goo(){return true}}");
}
public void testExponents() {
assertPrintNumber("1", 1);
assertPrintNumber("10", 10);
assertPrintNumber("100", 100);
assertPrintNumber("1E3", 1000);
assertPrintNumber("1E4", 10000);
assertPrintNumber("1E5", 100000);
assertPrintNumber("-1", -1);
assertPrintNumber("-10", -10);
assertPrintNumber("-100", -100);
assertPrintNumber("-1E3", -1000);
assertPrintNumber("-12341234E4", -123412340000L);
assertPrintNumber("1E18", 1000000000000000000L);
assertPrintNumber("1E5", 100000.0);
assertPrintNumber("100000.1", 100000.1);
assertPrintNumber("1E-6", 0.000001);
assertPrintNumber("-0x38d7ea4c68001", -0x38d7ea4c68001L);
assertPrintNumber("0x38d7ea4c68001", 0x38d7ea4c68001L);
}
// Make sure to test as both a String and a Node, because
// negative numbers do not parse consistently from strings.
private void assertPrintNumber(String expected, double number) {
assertPrint(String.valueOf(number), expected);
assertPrintNode(expected, Node.newNumber(number));
}
private void assertPrintNumber(String expected, int number) {
assertPrint(String.valueOf(number), expected);
assertPrintNode(expected, Node.newNumber(number));
}
public void testDirectEval() {
assertPrint("eval('1');", "eval(\"1\")");
}
public void testIndirectEval() {
Node n = parse("eval('1');");
assertPrintNode("eval(\"1\")", n);
n.getFirstChild().getFirstChild().getFirstChild().putBooleanProp(
Node.DIRECT_EVAL, false);
assertPrintNode("(0,eval)(\"1\")", n);
}
public void testFreeCall1() {
assertPrint("foo(a);", "foo(a)");
assertPrint("x.foo(a);", "x.foo(a)");
}
public void testFreeCall2() {
Node n = parse("foo(a);");
assertPrintNode("foo(a)", n);
Node call = n.getFirstChild().getFirstChild();
assertTrue(call.isCall());
call.putBooleanProp(Node.FREE_CALL, true);
assertPrintNode("foo(a)", n);
}
public void testFreeCall3() {
Node n = parse("x.foo(a);");
assertPrintNode("x.foo(a)", n);
Node call = n.getFirstChild().getFirstChild();
assertTrue(call.isCall());
call.putBooleanProp(Node.FREE_CALL, true);
assertPrintNode("(0,x.foo)(a)", n);
}
public void testPrintScript() {
// Verify that SCRIPT nodes not marked as synthetic are printed as
// blocks.
Node ast = new Node(Token.SCRIPT,
new Node(Token.EXPR_RESULT, Node.newString("f")),
new Node(Token.EXPR_RESULT, Node.newString("g")));
String result = new CodePrinter.Builder(ast).setPrettyPrint(true).build();
assertEquals("\"f\";\n\"g\";\n", result);
}
public void testObjectLit() {
assertPrint("({x:1})", "({x:1})");
assertPrint("var x=({x:1})", "var x={x:1}");
assertPrint("var x={'x':1}", "var x={\"x\":1}");
assertPrint("var x={1:1}", "var x={1:1}");
assertPrint("({},42)+0", "({},42)+0");
}
public void testObjectLit2() {
assertPrint("var x={1:1}", "var x={1:1}");
assertPrint("var x={'1':1}", "var x={1:1}");
assertPrint("var x={'1.0':1}", "var x={\"1.0\":1}");
assertPrint("var x={1.5:1}", "var x={\"1.5\":1}");
}
public void testObjectLit3() {
assertPrint("var x={3E9:1}",
"var x={3E9:1}");
assertPrint("var x={'3000000000':1}", // More than 31 bits
"var x={3E9:1}");
assertPrint("var x={'3000000001':1}",
"var x={3000000001:1}");
assertPrint("var x={'6000000001':1}", // More than 32 bits
"var x={6000000001:1}");
assertPrint("var x={\"12345678901234567\":1}", // More than 53 bits
"var x={\"12345678901234567\":1}");
}
public void testObjectLit4() {
// More than 128 bits.
assertPrint(
"var x={\"123456789012345671234567890123456712345678901234567\":1}",
"var x={\"123456789012345671234567890123456712345678901234567\":1}");
}
public void testGetter() {
assertPrint("var x = {}", "var x={}");
assertPrint("var x = {get a() {return 1}}", "var x={get a(){return 1}}");
assertPrint(
"var x = {get a() {}, get b(){}}",
"var x={get a(){},get b(){}}");
assertPrint(
"var x = {get 'a'() {return 1}}",
"var x={get \"a\"(){return 1}}");
assertPrint(
"var x = {get 1() {return 1}}",
"var x={get 1(){return 1}}");
assertPrint(
"var x = {get \"()\"() {return 1}}",
"var x={get \"()\"(){return 1}}");
languageMode = LanguageMode.ECMASCRIPT5;
assertPrintSame("var x={get function(){return 1}}");
// Getters and setters and not supported in ES3 but if someone sets the
// the ES3 output mode on an AST containing them we still produce them.
languageMode = LanguageMode.ECMASCRIPT3;
assertPrintSame("var x={get function(){return 1}}");
}
public void testSetter() {
assertPrint("var x = {}", "var x={}");
assertPrint(
"var x = {set a(y) {return 1}}",
"var x={set a(y){return 1}}");
assertPrint(
"var x = {get 'a'() {return 1}}",
"var x={get \"a\"(){return 1}}");
assertPrint(
"var x = {set 1(y) {return 1}}",
"var x={set 1(y){return 1}}");
assertPrint(
"var x = {set \"(x)\"(y) {return 1}}",
"var x={set \"(x)\"(y){return 1}}");
languageMode = LanguageMode.ECMASCRIPT5;
assertPrintSame("var x={set function(x){}}");
// Getters and setters and not supported in ES3 but if someone sets the
// the ES3 output mode on an AST containing them we still produce them.
languageMode = LanguageMode.ECMASCRIPT3;
assertPrintSame("var x={set function(x){}}");
}
public void testNegCollapse() {
// Collapse the negative symbol on numbers at generation time,
// to match the Rhino behavior.
assertPrint("var x = - - 2;", "var x=2");
assertPrint("var x = - (2);", "var x=-2");
}
public void testStrict() {
String result = parsePrint("var x", false, false, 0, false, true);
assertEquals("'use strict';var x", result);
}
public void testArrayLiteral() {
assertPrint("var x = [,];","var x=[,]");
assertPrint("var x = [,,];","var x=[,,]");
assertPrint("var x = [,s,,];","var x=[,s,,]");
assertPrint("var x = [,s];","var x=[,s]");
assertPrint("var x = [s,];","var x=[s]");
}
public void testZero() {
assertPrint("var x ='\\0';", "var x=\"\\x00\"");
assertPrint("var x ='\\x00';", "var x=\"\\x00\"");
assertPrint("var x ='\\u0000';", "var x=\"\\x00\"");
assertPrint("var x ='\\u00003';", "var x=\"\\x003\"");
}
public void testUnicode() {
assertPrint("var x ='\\x0f';", "var x=\"\\u000f\"");
assertPrint("var x ='\\x68';", "var x=\"h\"");
assertPrint("var x ='\\x7f';", "var x=\"\\u007f\"");
}
public void testUnicodeKeyword() {
// keyword "if"
assertPrint("var \\u0069\\u0066 = 1;", "var i\\u0066=1");
// keyword "var"
assertPrint("var v\\u0061\\u0072 = 1;", "var va\\u0072=1");
// all are keyword "while"
assertPrint("var w\\u0068\\u0069\\u006C\\u0065 = 1;"
+ "\\u0077\\u0068il\\u0065 = 2;"
+ "\\u0077h\\u0069le = 3;",
"var whil\\u0065=1;whil\\u0065=2;whil\\u0065=3");
}
public void testNumericKeys() {
assertPrint("var x = {010: 1};", "var x={8:1}");
assertPrint("var x = {'010': 1};", "var x={\"010\":1}");
assertPrint("var x = {0x10: 1};", "var x={16:1}");
assertPrint("var x = {'0x10': 1};", "var x={\"0x10\":1}");
// I was surprised at this result too.
assertPrint("var x = {.2: 1};", "var x={\"0.2\":1}");
assertPrint("var x = {'.2': 1};", "var x={\".2\":1}");
assertPrint("var x = {0.2: 1};", "var x={\"0.2\":1}");
assertPrint("var x = {'0.2': 1};", "var x={\"0.2\":1}");
}
public void testIssue582() {
assertPrint("var x = -0.0;", "var x=-0");
}
public void testIssue942() {
assertPrint("var x = {0: 1};", "var x={0:1}");
}
public void testIssue601() {
assertPrint("'\\v' == 'v'", "\"\\v\"==\"v\"");
assertPrint("'\\u000B' == '\\v'", "\"\\x0B\"==\"\\v\"");
assertPrint("'\\x0B' == '\\v'", "\"\\x0B\"==\"\\v\"");
}
public void testIssue620() {
assertPrint("alert(/ / / / /);", "alert(/ // / /)");
assertPrint("alert(/ // / /);", "alert(/ // / /)");
}
public void testIssue5746867() {
assertPrint("var a = { '$\\\\' : 5 };", "var a={\"$\\\\\":5}");
}
public void testCommaSpacing() {
assertPrint("var a = (b = 5, c = 5);",
"var a=(b=5,c=5)");
assertPrettyPrint("var a = (b = 5, c = 5);",
"var a = (b = 5, c = 5);\n");
}
public void testManyCommas() {
int numCommas = 10000;
List<String> numbers = Lists.newArrayList("0", "1");
Node current = new Node(Token.COMMA, Node.newNumber(0), Node.newNumber(1));
for (int i = 2; i < numCommas; i++) {
current = new Node(Token.COMMA, current);
// 1000 is printed as 1E3, and screws up our test.
int num = i % 1000;
numbers.add(String.valueOf(num));
current.addChildToBack(Node.newNumber(num));
}
String expected = Joiner.on(",").join(numbers);
String actual = printNode(current).replace("\n", "");
assertEquals(expected, actual);
}
public void testManyAdds() {
int numAdds = 10000;
List<String> numbers = Lists.newArrayList("0", "1");
Node current = new Node(Token.ADD, Node.newNumber(0), Node.newNumber(1));
for (int i = 2; i < numAdds; i++) {
current = new Node(Token.ADD, current);
// 1000 is printed as 1E3, and screws up our test.
int num = i % 1000;
numbers.add(String.valueOf(num));
current.addChildToBack(Node.newNumber(num));
}
String expected = Joiner.on("+").join(numbers);
String actual = printNode(current).replace("\n", "");
assertEquals(expected, actual);
}
public void testMinusNegativeZero() {
// Negative zero is weird, because we have to be able to distinguish
// it from positive zero (there are some subtle differences in behavior).
assertPrint("x- -0", "x- -0");
}
public void testStringEscapeSequences() {
// From the SingleEscapeCharacter grammar production.
assertPrintSame("var x=\"\\b\"");
assertPrintSame("var x=\"\\f\"");
assertPrintSame("var x=\"\\n\"");
assertPrintSame("var x=\"\\r\"");
assertPrintSame("var x=\"\\t\"");
assertPrintSame("var x=\"\\v\"");
assertPrint("var x=\"\\\"\"", "var x='\"'");
assertPrint("var x=\"\\\'\"", "var x=\"'\"");
// From the LineTerminator grammar
assertPrint("var x=\"\\u000A\"", "var x=\"\\n\"");
assertPrint("var x=\"\\u000D\"", "var x=\"\\r\"");
assertPrintSame("var x=\"\\u2028\"");
assertPrintSame("var x=\"\\u2029\"");
// Now with regular expressions.
assertPrintSame("var x=/\\b/");
assertPrintSame("var x=/\\f/");
assertPrintSame("var x=/\\n/");
assertPrintSame("var x=/\\r/");
assertPrintSame("var x=/\\t/");
assertPrintSame("var x=/\\v/");
assertPrintSame("var x=/\\u000A/");
assertPrintSame("var x=/\\u000D/");
assertPrintSame("var x=/\\u2028/");
assertPrintSame("var x=/\\u2029/");
}
public void testKeywordProperties1() {
languageMode = LanguageMode.ECMASCRIPT5;
assertPrintSame("x.foo=2");
assertPrintSame("x.function=2");
languageMode = LanguageMode.ECMASCRIPT3;
assertPrintSame("x.foo=2");
assertPrint("x.function=2", "x[\"function\"]=2");
}
public void testKeywordProperties2() {
languageMode = LanguageMode.ECMASCRIPT5;
assertPrintSame("x={foo:2}");
assertPrintSame("x={function:2}");
languageMode = LanguageMode.ECMASCRIPT3;
assertPrintSame("x={foo:2}");
assertPrint("x={function:2}", "x={\"function\":2}");
}
} | // You are a professional Java test case writer, please create a test case named `testPrintInOperatorInForLoop` for the issue `Closure-1033`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-1033
//
// ## Issue-Title:
// Generates code with invalid for/in left-hand assignment
//
// ## Issue-Description:
// **What steps will reproduce the problem?**
// 1. Compile this:
//
// window.Foo = function(A, B, C, D) {
// if ( A ) {
// if ( B ) {
// C = 0;
// } else {
// C = 0 in D;
// }
// while ( C-- ) {}
// }
// }
//
// **What is the expected output? What do you see instead?**
//
// Expected: Something that doesn't have a syntax error, maybe
//
// window.Foo=function(b,c,a,d){if(b)for(a=c?0:(0 in d);a--;);};
//
// Actual:
//
// window.Foo=function(b,c,a,d){if(b)for(a=c?0:0 in d;a--;);};
//
// SyntaxError: Unexpected token ; (Chrome)
// invalid for/in left-hand side (Firefox)
//
//
// **What version of the product are you using? On what operating system?**
//
// http://closure-compiler.appspot.com/home
//
//
// **Please provide any additional information below.**
//
// I noticed this while attempting to minify jquery
//
//
public void testPrintInOperatorInForLoop() {
| 471 | 123 | 438 | test/com/google/javascript/jscomp/CodePrinterTest.java | test | ```markdown
## Issue-ID: Closure-1033
## Issue-Title:
Generates code with invalid for/in left-hand assignment
## Issue-Description:
**What steps will reproduce the problem?**
1. Compile this:
window.Foo = function(A, B, C, D) {
if ( A ) {
if ( B ) {
C = 0;
} else {
C = 0 in D;
}
while ( C-- ) {}
}
}
**What is the expected output? What do you see instead?**
Expected: Something that doesn't have a syntax error, maybe
window.Foo=function(b,c,a,d){if(b)for(a=c?0:(0 in d);a--;);};
Actual:
window.Foo=function(b,c,a,d){if(b)for(a=c?0:0 in d;a--;);};
SyntaxError: Unexpected token ; (Chrome)
invalid for/in left-hand side (Firefox)
**What version of the product are you using? On what operating system?**
http://closure-compiler.appspot.com/home
**Please provide any additional information below.**
I noticed this while attempting to minify jquery
```
You are a professional Java test case writer, please create a test case named `testPrintInOperatorInForLoop` for the issue `Closure-1033`, utilizing the provided issue report information and the following function signature.
```java
public void testPrintInOperatorInForLoop() {
```
| 438 | [
"com.google.javascript.jscomp.CodeGenerator"
] | a3e67d98982693f3385f5d8609c8592dff343985595b8f770f1a5ca45556dbbb | public void testPrintInOperatorInForLoop() | // You are a professional Java test case writer, please create a test case named `testPrintInOperatorInForLoop` for the issue `Closure-1033`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Closure-1033
//
// ## Issue-Title:
// Generates code with invalid for/in left-hand assignment
//
// ## Issue-Description:
// **What steps will reproduce the problem?**
// 1. Compile this:
//
// window.Foo = function(A, B, C, D) {
// if ( A ) {
// if ( B ) {
// C = 0;
// } else {
// C = 0 in D;
// }
// while ( C-- ) {}
// }
// }
//
// **What is the expected output? What do you see instead?**
//
// Expected: Something that doesn't have a syntax error, maybe
//
// window.Foo=function(b,c,a,d){if(b)for(a=c?0:(0 in d);a--;);};
//
// Actual:
//
// window.Foo=function(b,c,a,d){if(b)for(a=c?0:0 in d;a--;);};
//
// SyntaxError: Unexpected token ; (Chrome)
// invalid for/in left-hand side (Firefox)
//
//
// **What version of the product are you using? On what operating system?**
//
// http://closure-compiler.appspot.com/home
//
//
// **Please provide any additional information below.**
//
// I noticed this while attempting to minify jquery
//
//
| Closure | /*
* Copyright 2004 The Closure Compiler Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.javascript.jscomp;
import com.google.common.base.Joiner;
import com.google.common.collect.Lists;
import com.google.javascript.jscomp.CompilerOptions.LanguageMode;
import com.google.javascript.rhino.InputId;
import com.google.javascript.rhino.Node;
import com.google.javascript.rhino.Token;
import junit.framework.TestCase;
import java.util.List;
public class CodePrinterTest extends TestCase {
private boolean trustedStrings = true;
private Compiler lastCompiler = null;
private LanguageMode languageMode = LanguageMode.ECMASCRIPT5;
@Override public void setUp() {
trustedStrings = true;
lastCompiler = null;
languageMode = LanguageMode.ECMASCRIPT5;
}
Node parse(String js) {
return parse(js, false);
}
Node parse(String js, boolean checkTypes) {
Compiler compiler = new Compiler();
lastCompiler = compiler;
CompilerOptions options = new CompilerOptions();
options.setTrustedStrings(trustedStrings);
// Allow getters and setters.
options.setLanguageIn(LanguageMode.ECMASCRIPT5);
compiler.initOptions(options);
Node n = compiler.parseTestCode(js);
if (checkTypes) {
DefaultPassConfig passConfig = new DefaultPassConfig(null);
CompilerPass typeResolver = passConfig.resolveTypes.create(compiler);
Node externs = new Node(Token.SCRIPT);
externs.setInputId(new InputId("externs"));
Node externAndJsRoot = new Node(Token.BLOCK, externs, n);
externAndJsRoot.setIsSyntheticBlock(true);
typeResolver.process(externs, n);
CompilerPass inferTypes = passConfig.inferTypes.create(compiler);
inferTypes.process(externs, n);
}
checkUnexpectedErrorsOrWarnings(compiler, 0);
return n;
}
private static void checkUnexpectedErrorsOrWarnings(
Compiler compiler, int expected) {
int actual = compiler.getErrors().length + compiler.getWarnings().length;
if (actual != expected) {
String msg = "";
for (JSError err : compiler.getErrors()) {
msg += "Error:" + err.toString() + "\n";
}
for (JSError err : compiler.getWarnings()) {
msg += "Warning:" + err.toString() + "\n";
}
assertEquals("Unexpected warnings or errors.\n " + msg, expected, actual);
}
}
String parsePrint(String js, boolean prettyprint, int lineThreshold) {
CompilerOptions options = new CompilerOptions();
options.setTrustedStrings(trustedStrings);
options.setPrettyPrint(prettyprint);
options.setLineLengthThreshold(lineThreshold);
options.setLanguageOut(languageMode);
return new CodePrinter.Builder(parse(js)).setCompilerOptions(options)
.build();
}
String parsePrint(String js, boolean prettyprint, boolean lineBreak,
int lineThreshold) {
CompilerOptions options = new CompilerOptions();
options.setTrustedStrings(trustedStrings);
options.setPrettyPrint(prettyprint);
options.setLineLengthThreshold(lineThreshold);
options.setLineBreak(lineBreak);
options.setLanguageOut(languageMode);
return new CodePrinter.Builder(parse(js)).setCompilerOptions(options)
.build();
}
String parsePrint(String js, boolean prettyprint, boolean lineBreak,
boolean preferLineBreakAtEof, int lineThreshold) {
CompilerOptions options = new CompilerOptions();
options.setTrustedStrings(trustedStrings);
options.setPrettyPrint(prettyprint);
options.setLineLengthThreshold(lineThreshold);
options.setPreferLineBreakAtEndOfFile(preferLineBreakAtEof);
options.setLineBreak(lineBreak);
options.setLanguageOut(languageMode);
return new CodePrinter.Builder(parse(js)).setCompilerOptions(options)
.build();
}
String parsePrint(String js, boolean prettyprint, boolean lineBreak,
int lineThreshold, boolean outputTypes) {
Node node = parse(js, true);
CompilerOptions options = new CompilerOptions();
options.setTrustedStrings(trustedStrings);
options.setPrettyPrint(prettyprint);
options.setLineLengthThreshold(lineThreshold);
options.setLineBreak(lineBreak);
options.setLanguageOut(languageMode);
return new CodePrinter.Builder(node).setCompilerOptions(options)
.setOutputTypes(outputTypes)
.setTypeRegistry(lastCompiler.getTypeRegistry())
.build();
}
String parsePrint(String js, boolean prettyprint, boolean lineBreak,
int lineThreshold, boolean outputTypes,
boolean tagAsStrict) {
Node node = parse(js, true);
CompilerOptions options = new CompilerOptions();
options.setTrustedStrings(trustedStrings);
options.setPrettyPrint(prettyprint);
options.setLineLengthThreshold(lineThreshold);
options.setLineBreak(lineBreak);
options.setLanguageOut(languageMode);
return new CodePrinter.Builder(node).setCompilerOptions(options)
.setOutputTypes(outputTypes)
.setTypeRegistry(lastCompiler.getTypeRegistry())
.setTagAsStrict(tagAsStrict)
.build();
}
String printNode(Node n) {
CompilerOptions options = new CompilerOptions();
options.setLineLengthThreshold(CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD);
options.setLanguageOut(languageMode);
return new CodePrinter.Builder(n).setCompilerOptions(options).build();
}
void assertPrintNode(String expectedJs, Node ast) {
assertEquals(expectedJs, printNode(ast));
}
public void testPrint() {
assertPrint("10 + a + b", "10+a+b");
assertPrint("10 + (30*50)", "10+30*50");
assertPrint("with(x) { x + 3; }", "with(x)x+3");
assertPrint("\"aa'a\"", "\"aa'a\"");
assertPrint("\"aa\\\"a\"", "'aa\"a'");
assertPrint("function foo()\n{return 10;}", "function foo(){return 10}");
assertPrint("a instanceof b", "a instanceof b");
assertPrint("typeof(a)", "typeof a");
assertPrint(
"var foo = x ? { a : 1 } : {a: 3, b:4, \"default\": 5, \"foo-bar\": 6}",
"var foo=x?{a:1}:{a:3,b:4,\"default\":5,\"foo-bar\":6}");
// Safari: needs ';' at the end of a throw statement
assertPrint("function foo(){throw 'error';}",
"function foo(){throw\"error\";}");
// Safari 3 needs a "{" around a single function
assertPrint("if (true) function foo(){return}",
"if(true){function foo(){return}}");
assertPrint("var x = 10; { var y = 20; }", "var x=10;var y=20");
assertPrint("while (x-- > 0);", "while(x-- >0);");
assertPrint("x-- >> 1", "x-- >>1");
assertPrint("(function () {})(); ",
"(function(){})()");
// Associativity
assertPrint("var a,b,c,d;a || (b&& c) && (a || d)",
"var a,b,c,d;a||b&&c&&(a||d)");
assertPrint("var a,b,c; a || (b || c); a * (b * c); a | (b | c)",
"var a,b,c;a||b||c;a*b*c;a|b|c");
assertPrint("var a,b,c; a / b / c;a / (b / c); a - (b - c);",
"var a,b,c;a/b/c;a/(b/c);a-(b-c)");
assertPrint("var a,b; a = b = 3;",
"var a,b;a=b=3");
assertPrint("var a,b,c,d; a = (b = c = (d = 3));",
"var a,b,c,d;a=b=c=d=3");
assertPrint("var a,b,c; a += (b = c += 3);",
"var a,b,c;a+=b=c+=3");
assertPrint("var a,b,c; a *= (b -= c);",
"var a,b,c;a*=b-=c");
// Precedence
assertPrint("a ? delete b[0] : 3", "a?delete b[0]:3");
assertPrint("(delete a[0])/10", "delete a[0]/10");
// optional '()' for new
// simple new
assertPrint("new A", "new A");
assertPrint("new A()", "new A");
assertPrint("new A('x')", "new A(\"x\")");
// calling instance method directly after new
assertPrint("new A().a()", "(new A).a()");
assertPrint("(new A).a()", "(new A).a()");
// this case should be fixed
assertPrint("new A('y').a()", "(new A(\"y\")).a()");
// internal class
assertPrint("new A.B", "new A.B");
assertPrint("new A.B()", "new A.B");
assertPrint("new A.B('z')", "new A.B(\"z\")");
// calling instance method directly after new internal class
assertPrint("(new A.B).a()", "(new A.B).a()");
assertPrint("new A.B().a()", "(new A.B).a()");
// this case should be fixed
assertPrint("new A.B('w').a()", "(new A.B(\"w\")).a()");
// Operators: make sure we don't convert binary + and unary + into ++
assertPrint("x + +y", "x+ +y");
assertPrint("x - (-y)", "x- -y");
assertPrint("x++ +y", "x++ +y");
assertPrint("x-- -y", "x-- -y");
assertPrint("x++ -y", "x++-y");
// Label
assertPrint("foo:for(;;){break foo;}", "foo:for(;;)break foo");
assertPrint("foo:while(1){continue foo;}", "foo:while(1)continue foo");
// Object literals.
assertPrint("({})", "({})");
assertPrint("var x = {};", "var x={}");
assertPrint("({}).x", "({}).x");
assertPrint("({})['x']", "({})[\"x\"]");
assertPrint("({}) instanceof Object", "({})instanceof Object");
assertPrint("({}) || 1", "({})||1");
assertPrint("1 || ({})", "1||{}");
assertPrint("({}) ? 1 : 2", "({})?1:2");
assertPrint("0 ? ({}) : 2", "0?{}:2");
assertPrint("0 ? 1 : ({})", "0?1:{}");
assertPrint("typeof ({})", "typeof{}");
assertPrint("f({})", "f({})");
// Anonymous function expressions.
assertPrint("(function(){})", "(function(){})");
assertPrint("(function(){})()", "(function(){})()");
assertPrint("(function(){})instanceof Object",
"(function(){})instanceof Object");
assertPrint("(function(){}).bind().call()",
"(function(){}).bind().call()");
assertPrint("var x = function() { };", "var x=function(){}");
assertPrint("var x = function() { }();", "var x=function(){}()");
assertPrint("(function() {}), 2", "(function(){}),2");
// Name functions expression.
assertPrint("(function f(){})", "(function f(){})");
// Function declaration.
assertPrint("function f(){}", "function f(){}");
// Make sure we don't treat non-Latin character escapes as raw strings.
assertPrint("({ 'a': 4, '\\u0100': 4 })", "({\"a\":4,\"\\u0100\":4})");
assertPrint("({ a: 4, '\\u0100': 4 })", "({a:4,\"\\u0100\":4})");
// Test if statement and for statements with single statements in body.
assertPrint("if (true) { alert();}", "if(true)alert()");
assertPrint("if (false) {} else {alert(\"a\");}",
"if(false);else alert(\"a\")");
assertPrint("for(;;) { alert();};", "for(;;)alert()");
assertPrint("do { alert(); } while(true);",
"do alert();while(true)");
assertPrint("myLabel: { alert();}",
"myLabel:alert()");
assertPrint("myLabel: for(;;) continue myLabel;",
"myLabel:for(;;)continue myLabel");
// Test nested var statement
assertPrint("if (true) var x; x = 4;", "if(true)var x;x=4");
// Non-latin identifier. Make sure we keep them escaped.
assertPrint("\\u00fb", "\\u00fb");
assertPrint("\\u00fa=1", "\\u00fa=1");
assertPrint("function \\u00f9(){}", "function \\u00f9(){}");
assertPrint("x.\\u00f8", "x.\\u00f8");
assertPrint("x.\\u00f8", "x.\\u00f8");
assertPrint("abc\\u4e00\\u4e01jkl", "abc\\u4e00\\u4e01jkl");
// Test the right-associative unary operators for spurious parens
assertPrint("! ! true", "!!true");
assertPrint("!(!(true))", "!!true");
assertPrint("typeof(void(0))", "typeof void 0");
assertPrint("typeof(void(!0))", "typeof void!0");
assertPrint("+ - + + - + 3", "+-+ +-+3"); // chained unary plus/minus
assertPrint("+(--x)", "+--x");
assertPrint("-(++x)", "-++x");
// needs a space to prevent an ambiguous parse
assertPrint("-(--x)", "- --x");
assertPrint("!(~~5)", "!~~5");
assertPrint("~(a/b)", "~(a/b)");
// Preserve parens to overcome greedy binding of NEW
assertPrint("new (foo.bar()).factory(baz)", "new (foo.bar().factory)(baz)");
assertPrint("new (bar()).factory(baz)", "new (bar().factory)(baz)");
assertPrint("new (new foobar(x)).factory(baz)",
"new (new foobar(x)).factory(baz)");
// Make sure that HOOK is right associative
assertPrint("a ? b : (c ? d : e)", "a?b:c?d:e");
assertPrint("a ? (b ? c : d) : e", "a?b?c:d:e");
assertPrint("(a ? b : c) ? d : e", "(a?b:c)?d:e");
// Test nested ifs
assertPrint("if (x) if (y); else;", "if(x)if(y);else;");
// Test comma.
assertPrint("a,b,c", "a,b,c");
assertPrint("(a,b),c", "a,b,c");
assertPrint("a,(b,c)", "a,b,c");
assertPrint("x=a,b,c", "x=a,b,c");
assertPrint("x=(a,b),c", "x=(a,b),c");
assertPrint("x=a,(b,c)", "x=a,b,c");
assertPrint("x=a,y=b,z=c", "x=a,y=b,z=c");
assertPrint("x=(a,y=b,z=c)", "x=(a,y=b,z=c)");
assertPrint("x=[a,b,c,d]", "x=[a,b,c,d]");
assertPrint("x=[(a,b,c),d]", "x=[(a,b,c),d]");
assertPrint("x=[(a,(b,c)),d]", "x=[(a,b,c),d]");
assertPrint("x=[a,(b,c,d)]", "x=[a,(b,c,d)]");
assertPrint("var x=(a,b)", "var x=(a,b)");
assertPrint("var x=a,b,c", "var x=a,b,c");
assertPrint("var x=(a,b),c", "var x=(a,b),c");
assertPrint("var x=a,b=(c,d)", "var x=a,b=(c,d)");
assertPrint("foo(a,b,c,d)", "foo(a,b,c,d)");
assertPrint("foo((a,b,c),d)", "foo((a,b,c),d)");
assertPrint("foo((a,(b,c)),d)", "foo((a,b,c),d)");
assertPrint("f(a+b,(c,d,(e,f,g)))", "f(a+b,(c,d,e,f,g))");
assertPrint("({}) , 1 , 2", "({}),1,2");
assertPrint("({}) , {} , {}", "({}),{},{}");
// EMPTY nodes
assertPrint("if (x){}", "if(x);");
assertPrint("if(x);", "if(x);");
assertPrint("if(x)if(y);", "if(x)if(y);");
assertPrint("if(x){if(y);}", "if(x)if(y);");
assertPrint("if(x){if(y){};;;}", "if(x)if(y);");
assertPrint("if(x){;;function y(){};;}", "if(x){function y(){}}");
}
public void testBreakTrustedStrings() {
// Break scripts
assertPrint("'<script>'", "\"<script>\"");
assertPrint("'</script>'", "\"\\x3c/script>\"");
assertPrint("\"</script> </SCRIPT>\"", "\"\\x3c/script> \\x3c/SCRIPT>\"");
assertPrint("'-->'", "\"--\\x3e\"");
assertPrint("']]>'", "\"]]\\x3e\"");
assertPrint("' --></script>'", "\" --\\x3e\\x3c/script>\"");
assertPrint("/--> <\\/script>/g", "/--\\x3e <\\/script>/g");
// Break HTML start comments. Certain versions of WebKit
// begin an HTML comment when they see this.
assertPrint("'<!-- I am a string -->'",
"\"\\x3c!-- I am a string --\\x3e\"");
assertPrint("'<=&>'", "\"<=&>\"");
}
public void testBreakUntrustedStrings() {
trustedStrings = false;
// Break scripts
assertPrint("'<script>'", "\"\\x3cscript\\x3e\"");
assertPrint("'</script>'", "\"\\x3c/script\\x3e\"");
assertPrint("\"</script> </SCRIPT>\"", "\"\\x3c/script\\x3e \\x3c/SCRIPT\\x3e\"");
assertPrint("'-->'", "\"--\\x3e\"");
assertPrint("']]>'", "\"]]\\x3e\"");
assertPrint("' --></script>'", "\" --\\x3e\\x3c/script\\x3e\"");
assertPrint("/--> <\\/script>/g", "/--\\x3e <\\/script>/g");
// Break HTML start comments. Certain versions of WebKit
// begin an HTML comment when they see this.
assertPrint("'<!-- I am a string -->'",
"\"\\x3c!-- I am a string --\\x3e\"");
assertPrint("'<=&>'", "\"\\x3c\\x3d\\x26\\x3e\"");
assertPrint("/(?=x)/", "/(?=x)/");
}
public void testPrintArray() {
assertPrint("[void 0, void 0]", "[void 0,void 0]");
assertPrint("[undefined, undefined]", "[undefined,undefined]");
assertPrint("[ , , , undefined]", "[,,,undefined]");
assertPrint("[ , , , 0]", "[,,,0]");
}
public void testHook() {
assertPrint("a ? b = 1 : c = 2", "a?b=1:c=2");
assertPrint("x = a ? b = 1 : c = 2", "x=a?b=1:c=2");
assertPrint("(x = a) ? b = 1 : c = 2", "(x=a)?b=1:c=2");
assertPrint("x, a ? b = 1 : c = 2", "x,a?b=1:c=2");
assertPrint("x, (a ? b = 1 : c = 2)", "x,a?b=1:c=2");
assertPrint("(x, a) ? b = 1 : c = 2", "(x,a)?b=1:c=2");
assertPrint("a ? (x, b) : c = 2", "a?(x,b):c=2");
assertPrint("a ? b = 1 : (x,c)", "a?b=1:(x,c)");
assertPrint("a ? b = 1 : c = 2 + x", "a?b=1:c=2+x");
assertPrint("(a ? b = 1 : c = 2) + x", "(a?b=1:c=2)+x");
assertPrint("a ? b = 1 : (c = 2) + x", "a?b=1:(c=2)+x");
assertPrint("a ? (b?1:2) : 3", "a?b?1:2:3");
}
public void testPrintInOperatorInForLoop() {
// Check for in expression in for's init expression.
// Check alone, with + (higher precedence), with ?: (lower precedence),
// and with conditional.
assertPrint("var a={}; for (var i = (\"length\" in a); i;) {}",
"var a={};for(var i=(\"length\"in a);i;);");
assertPrint("var a={}; for (var i = (\"length\" in a) ? 0 : 1; i;) {}",
"var a={};for(var i=(\"length\"in a)?0:1;i;);");
assertPrint("var a={}; for (var i = (\"length\" in a) + 1; i;) {}",
"var a={};for(var i=(\"length\"in a)+1;i;);");
assertPrint("var a={};for (var i = (\"length\" in a|| \"size\" in a);;);",
"var a={};for(var i=(\"length\"in a)||(\"size\"in a);;);");
assertPrint("var a={};for (var i = a || a || (\"size\" in a);;);",
"var a={};for(var i=a||a||(\"size\"in a);;);");
// Test works with unary operators and calls.
assertPrint("var a={}; for (var i = -(\"length\" in a); i;) {}",
"var a={};for(var i=-(\"length\"in a);i;);");
assertPrint("var a={};function b_(p){ return p;};" +
"for(var i=1,j=b_(\"length\" in a);;) {}",
"var a={};function b_(p){return p}" +
"for(var i=1,j=b_(\"length\"in a);;);");
// Test we correctly handle an in operator in the test clause.
assertPrint("var a={}; for (;(\"length\" in a);) {}",
"var a={};for(;\"length\"in a;);");
// Test we correctly handle an in operator inside a comma.
assertPrintSame("for(x,(y in z);;)foo()");
assertPrintSame("for(var x,w=(y in z);;)foo()");
// And in operator inside a hook.
assertPrintSame("for(a=c?0:(0 in d);;)foo()");
}
public void testLiteralProperty() {
assertPrint("(64).toString()", "(64).toString()");
}
private void assertPrint(String js, String expected) {
parse(expected); // validate the expected string is valid JS
assertEquals(expected,
parsePrint(js, false, CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD));
}
private void assertPrintSame(String js) {
assertPrint(js, js);
}
// Make sure that the code generator doesn't associate an
// else clause with the wrong if clause.
public void testAmbiguousElseClauses() {
assertPrintNode("if(x)if(y);else;",
new Node(Token.IF,
Node.newString(Token.NAME, "x"),
new Node(Token.BLOCK,
new Node(Token.IF,
Node.newString(Token.NAME, "y"),
new Node(Token.BLOCK),
// ELSE clause for the inner if
new Node(Token.BLOCK)))));
assertPrintNode("if(x){if(y);}else;",
new Node(Token.IF,
Node.newString(Token.NAME, "x"),
new Node(Token.BLOCK,
new Node(Token.IF,
Node.newString(Token.NAME, "y"),
new Node(Token.BLOCK))),
// ELSE clause for the outer if
new Node(Token.BLOCK)));
assertPrintNode("if(x)if(y);else{if(z);}else;",
new Node(Token.IF,
Node.newString(Token.NAME, "x"),
new Node(Token.BLOCK,
new Node(Token.IF,
Node.newString(Token.NAME, "y"),
new Node(Token.BLOCK),
new Node(Token.BLOCK,
new Node(Token.IF,
Node.newString(Token.NAME, "z"),
new Node(Token.BLOCK))))),
// ELSE clause for the outermost if
new Node(Token.BLOCK)));
}
public void testLineBreak() {
// line break after function if in a statement context
assertLineBreak("function a() {}\n" +
"function b() {}",
"function a(){}\n" +
"function b(){}\n");
// line break after ; after a function
assertLineBreak("var a = {};\n" +
"a.foo = function () {}\n" +
"function b() {}",
"var a={};a.foo=function(){};\n" +
"function b(){}\n");
// break after comma after a function
assertLineBreak("var a = {\n" +
" b: function() {},\n" +
" c: function() {}\n" +
"};\n" +
"alert(a);",
"var a={b:function(){},\n" +
"c:function(){}};\n" +
"alert(a)");
}
private void assertLineBreak(String js, String expected) {
assertEquals(expected,
parsePrint(js, false, true,
CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD));
}
public void testPreferLineBreakAtEndOfFile() {
// short final line, no previous break, do nothing
assertLineBreakAtEndOfFile(
"\"1234567890\";",
"\"1234567890\"",
"\"1234567890\"");
// short final line, shift previous break to end
assertLineBreakAtEndOfFile(
"\"123456789012345678901234567890\";\"1234567890\"",
"\"123456789012345678901234567890\";\n\"1234567890\"",
"\"123456789012345678901234567890\"; \"1234567890\";\n");
assertLineBreakAtEndOfFile(
"var12345678901234567890123456 instanceof Object;",
"var12345678901234567890123456 instanceof\nObject",
"var12345678901234567890123456 instanceof Object;\n");
// long final line, no previous break, add a break at end
assertLineBreakAtEndOfFile(
"\"1234567890\";\"12345678901234567890\";",
"\"1234567890\";\"12345678901234567890\"",
"\"1234567890\";\"12345678901234567890\";\n");
// long final line, previous break, add a break at end
assertLineBreakAtEndOfFile(
"\"123456789012345678901234567890\";\"12345678901234567890\";",
"\"123456789012345678901234567890\";\n\"12345678901234567890\"",
"\"123456789012345678901234567890\";\n\"12345678901234567890\";\n");
}
private void assertLineBreakAtEndOfFile(String js,
String expectedWithoutBreakAtEnd, String expectedWithBreakAtEnd) {
assertEquals(expectedWithoutBreakAtEnd,
parsePrint(js, false, false, false, 30));
assertEquals(expectedWithBreakAtEnd,
parsePrint(js, false, false, true, 30));
}
public void testPrettyPrinter() {
// Ensure that the pretty printer inserts line breaks at appropriate
// places.
assertPrettyPrint("(function(){})();","(function() {\n})();\n");
assertPrettyPrint("var a = (function() {});alert(a);",
"var a = function() {\n};\nalert(a);\n");
// Check we correctly handle putting brackets around all if clauses so
// we can put breakpoints inside statements.
assertPrettyPrint("if (1) {}",
"if(1) {\n" +
"}\n");
assertPrettyPrint("if (1) {alert(\"\");}",
"if(1) {\n" +
" alert(\"\")\n" +
"}\n");
assertPrettyPrint("if (1)alert(\"\");",
"if(1) {\n" +
" alert(\"\")\n" +
"}\n");
assertPrettyPrint("if (1) {alert();alert();}",
"if(1) {\n" +
" alert();\n" +
" alert()\n" +
"}\n");
// Don't add blocks if they weren't there already.
assertPrettyPrint("label: alert();",
"label:alert();\n");
// But if statements and loops get blocks automagically.
assertPrettyPrint("if (1) alert();",
"if(1) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint("for (;;) alert();",
"for(;;) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint("while (1) alert();",
"while(1) {\n" +
" alert()\n" +
"}\n");
// Do we put else clauses in blocks?
assertPrettyPrint("if (1) {} else {alert(a);}",
"if(1) {\n" +
"}else {\n alert(a)\n}\n");
// Do we add blocks to else clauses?
assertPrettyPrint("if (1) alert(a); else alert(b);",
"if(1) {\n" +
" alert(a)\n" +
"}else {\n" +
" alert(b)\n" +
"}\n");
// Do we put for bodies in blocks?
assertPrettyPrint("for(;;) { alert();}",
"for(;;) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint("for(;;) {}",
"for(;;) {\n" +
"}\n");
assertPrettyPrint("for(;;) { alert(); alert(); }",
"for(;;) {\n" +
" alert();\n" +
" alert()\n" +
"}\n");
// How about do loops?
assertPrettyPrint("do { alert(); } while(true);",
"do {\n" +
" alert()\n" +
"}while(true);\n");
// label?
assertPrettyPrint("myLabel: { alert();}",
"myLabel: {\n" +
" alert()\n" +
"}\n");
// Don't move the label on a loop, because then break {label} and
// continue {label} won't work.
assertPrettyPrint("myLabel: for(;;) continue myLabel;",
"myLabel:for(;;) {\n" +
" continue myLabel\n" +
"}\n");
assertPrettyPrint("var a;", "var a;\n");
}
public void testPrettyPrinter2() {
assertPrettyPrint(
"if(true) f();",
"if(true) {\n" +
" f()\n" +
"}\n");
assertPrettyPrint(
"if (true) { f() } else { g() }",
"if(true) {\n" +
" f()\n" +
"}else {\n" +
" g()\n" +
"}\n");
assertPrettyPrint(
"if(true) f(); for(;;) g();",
"if(true) {\n" +
" f()\n" +
"}\n" +
"for(;;) {\n" +
" g()\n" +
"}\n");
}
public void testPrettyPrinter3() {
assertPrettyPrint(
"try {} catch(e) {}if (1) {alert();alert();}",
"try {\n" +
"}catch(e) {\n" +
"}\n" +
"if(1) {\n" +
" alert();\n" +
" alert()\n" +
"}\n");
assertPrettyPrint(
"try {} finally {}if (1) {alert();alert();}",
"try {\n" +
"}finally {\n" +
"}\n" +
"if(1) {\n" +
" alert();\n" +
" alert()\n" +
"}\n");
assertPrettyPrint(
"try {} catch(e) {} finally {} if (1) {alert();alert();}",
"try {\n" +
"}catch(e) {\n" +
"}finally {\n" +
"}\n" +
"if(1) {\n" +
" alert();\n" +
" alert()\n" +
"}\n");
}
public void testPrettyPrinter4() {
assertPrettyPrint(
"function f() {}if (1) {alert();}",
"function f() {\n" +
"}\n" +
"if(1) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint(
"var f = function() {};if (1) {alert();}",
"var f = function() {\n" +
"};\n" +
"if(1) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint(
"(function() {})();if (1) {alert();}",
"(function() {\n" +
"})();\n" +
"if(1) {\n" +
" alert()\n" +
"}\n");
assertPrettyPrint(
"(function() {alert();alert();})();if (1) {alert();}",
"(function() {\n" +
" alert();\n" +
" alert()\n" +
"})();\n" +
"if(1) {\n" +
" alert()\n" +
"}\n");
}
public void testTypeAnnotations() {
assertTypeAnnotations(
"/** @constructor */ function Foo(){}",
"/**\n * @return {undefined}\n * @constructor\n */\n"
+ "function Foo() {\n}\n");
}
public void testTypeAnnotationsTypeDef() {
// TODO(johnlenz): It would be nice if there were some way to preserve
// typedefs but currently they are resolved into the basic types in the
// type registry.
assertTypeAnnotations(
"/** @typedef {Array.<number>} */ goog.java.Long;\n"
+ "/** @param {!goog.java.Long} a*/\n"
+ "function f(a){};\n",
"goog.java.Long;\n"
+ "/**\n"
+ " * @param {(Array.<number>|null)} a\n"
+ " * @return {undefined}\n"
+ " */\n"
+ "function f(a) {\n}\n");
}
public void testTypeAnnotationsAssign() {
assertTypeAnnotations("/** @constructor */ var Foo = function(){}",
"/**\n * @return {undefined}\n * @constructor\n */\n"
+ "var Foo = function() {\n};\n");
}
public void testTypeAnnotationsNamespace() {
assertTypeAnnotations("var a = {};"
+ "/** @constructor */ a.Foo = function(){}",
"var a = {};\n"
+ "/**\n * @return {undefined}\n * @constructor\n */\n"
+ "a.Foo = function() {\n};\n");
}
public void testTypeAnnotationsMemberSubclass() {
assertTypeAnnotations("var a = {};"
+ "/** @constructor */ a.Foo = function(){};"
+ "/** @constructor \n @extends {a.Foo} */ a.Bar = function(){}",
"var a = {};\n"
+ "/**\n * @return {undefined}\n * @constructor\n */\n"
+ "a.Foo = function() {\n};\n"
+ "/**\n * @return {undefined}\n * @extends {a.Foo}\n"
+ " * @constructor\n */\n"
+ "a.Bar = function() {\n};\n");
}
public void testTypeAnnotationsInterface() {
assertTypeAnnotations("var a = {};"
+ "/** @interface */ a.Foo = function(){};"
+ "/** @interface \n @extends {a.Foo} */ a.Bar = function(){}",
"var a = {};\n"
+ "/**\n * @interface\n */\n"
+ "a.Foo = function() {\n};\n"
+ "/**\n * @extends {a.Foo}\n"
+ " * @interface\n */\n"
+ "a.Bar = function() {\n};\n");
}
public void testTypeAnnotationsMultipleInterface() {
assertTypeAnnotations("var a = {};"
+ "/** @interface */ a.Foo1 = function(){};"
+ "/** @interface */ a.Foo2 = function(){};"
+ "/** @interface \n @extends {a.Foo1} \n @extends {a.Foo2} */"
+ "a.Bar = function(){}",
"var a = {};\n"
+ "/**\n * @interface\n */\n"
+ "a.Foo1 = function() {\n};\n"
+ "/**\n * @interface\n */\n"
+ "a.Foo2 = function() {\n};\n"
+ "/**\n * @extends {a.Foo1}\n"
+ " * @extends {a.Foo2}\n"
+ " * @interface\n */\n"
+ "a.Bar = function() {\n};\n");
}
public void testTypeAnnotationsMember() {
assertTypeAnnotations("var a = {};"
+ "/** @constructor */ a.Foo = function(){}"
+ "/** @param {string} foo\n"
+ " * @return {number} */\n"
+ "a.Foo.prototype.foo = function(foo) { return 3; };"
+ "/** @type {string|undefined} */"
+ "a.Foo.prototype.bar = '';",
"var a = {};\n"
+ "/**\n * @return {undefined}\n * @constructor\n */\n"
+ "a.Foo = function() {\n};\n"
+ "/**\n"
+ " * @param {string} foo\n"
+ " * @return {number}\n"
+ " */\n"
+ "a.Foo.prototype.foo = function(foo) {\n return 3\n};\n"
+ "/** @type {string} */\n"
+ "a.Foo.prototype.bar = \"\";\n");
}
public void testTypeAnnotationsImplements() {
assertTypeAnnotations("var a = {};"
+ "/** @constructor */ a.Foo = function(){};\n"
+ "/** @interface */ a.I = function(){};\n"
+ "/** @interface */ a.I2 = function(){};\n"
+ "/** @constructor \n @extends {a.Foo}\n"
+ " * @implements {a.I} \n @implements {a.I2}\n"
+ "*/ a.Bar = function(){}",
"var a = {};\n"
+ "/**\n * @return {undefined}\n * @constructor\n */\n"
+ "a.Foo = function() {\n};\n"
+ "/**\n * @interface\n */\n"
+ "a.I = function() {\n};\n"
+ "/**\n * @interface\n */\n"
+ "a.I2 = function() {\n};\n"
+ "/**\n * @return {undefined}\n * @extends {a.Foo}\n"
+ " * @implements {a.I}\n"
+ " * @implements {a.I2}\n * @constructor\n */\n"
+ "a.Bar = function() {\n};\n");
}
public void testTypeAnnotationsDispatcher1() {
assertTypeAnnotations(
"var a = {};\n" +
"/** \n" +
" * @constructor \n" +
" * @javadispatch \n" +
" */\n" +
"a.Foo = function(){}",
"var a = {};\n" +
"/**\n" +
" * @return {undefined}\n" +
" * @constructor\n" +
" * @javadispatch\n" +
" */\n" +
"a.Foo = function() {\n" +
"};\n");
}
public void testTypeAnnotationsDispatcher2() {
assertTypeAnnotations(
"var a = {};\n" +
"/** \n" +
" * @constructor \n" +
" */\n" +
"a.Foo = function(){}\n" +
"/**\n" +
" * @javadispatch\n" +
" */\n" +
"a.Foo.prototype.foo = function() {};",
"var a = {};\n" +
"/**\n" +
" * @return {undefined}\n" +
" * @constructor\n" +
" */\n" +
"a.Foo = function() {\n" +
"};\n" +
"/**\n" +
" * @return {undefined}\n" +
" * @javadispatch\n" +
" */\n" +
"a.Foo.prototype.foo = function() {\n" +
"};\n");
}
public void testU2UFunctionTypeAnnotation1() {
assertTypeAnnotations(
"/** @type {!Function} */ var x = function() {}",
"/** @type {!Function} */\n" +
"var x = function() {\n};\n");
}
public void testU2UFunctionTypeAnnotation2() {
// TODO(johnlenz): we currently report the type of the RHS which is not
// correct, we should export the type of the LHS.
assertTypeAnnotations(
"/** @type {Function} */ var x = function() {}",
"/** @type {!Function} */\n" +
"var x = function() {\n};\n");
}
public void testEmitUnknownParamTypesAsAllType() {
assertTypeAnnotations(
"var a = function(x) {}",
"/**\n" +
" * @param {?} x\n" +
" * @return {undefined}\n" +
" */\n" +
"var a = function(x) {\n};\n");
}
public void testOptionalTypesAnnotation() {
assertTypeAnnotations(
"/**\n" +
" * @param {string=} x \n" +
" */\n" +
"var a = function(x) {}",
"/**\n" +
" * @param {string=} x\n" +
" * @return {undefined}\n" +
" */\n" +
"var a = function(x) {\n};\n");
}
public void testVariableArgumentsTypesAnnotation() {
assertTypeAnnotations(
"/**\n" +
" * @param {...string} x \n" +
" */\n" +
"var a = function(x) {}",
"/**\n" +
" * @param {...string} x\n" +
" * @return {undefined}\n" +
" */\n" +
"var a = function(x) {\n};\n");
}
public void testTempConstructor() {
assertTypeAnnotations(
"var x = function() {\n/**\n * @constructor\n */\nfunction t1() {}\n" +
" /**\n * @constructor\n */\nfunction t2() {}\n" +
" t1.prototype = t2.prototype}",
"/**\n * @return {undefined}\n */\nvar x = function() {\n" +
" /**\n * @return {undefined}\n * @constructor\n */\n" +
"function t1() {\n }\n" +
" /**\n * @return {undefined}\n * @constructor\n */\n" +
"function t2() {\n }\n" +
" t1.prototype = t2.prototype\n};\n"
);
}
public void testEnumAnnotation1() {
assertTypeAnnotations(
"/** @enum {string} */ var Enum = {FOO: 'x', BAR: 'y'};",
"/** @enum {string} */\nvar Enum = {FOO:\"x\", BAR:\"y\"};\n");
}
public void testEnumAnnotation2() {
assertTypeAnnotations(
"var goog = goog || {};" +
"/** @enum {string} */ goog.Enum = {FOO: 'x', BAR: 'y'};" +
"/** @const */ goog.Enum2 = goog.x ? {} : goog.Enum;",
"var goog = goog || {};\n" +
"/** @enum {string} */\ngoog.Enum = {FOO:\"x\", BAR:\"y\"};\n" +
"/** @type {(Object|{})} */\ngoog.Enum2 = goog.x ? {} : goog.Enum;\n");
}
private void assertPrettyPrint(String js, String expected) {
assertEquals(expected,
parsePrint(js, true, false,
CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD));
}
private void assertTypeAnnotations(String js, String expected) {
assertEquals(expected,
parsePrint(js, true, false,
CodePrinter.DEFAULT_LINE_LENGTH_THRESHOLD, true));
}
public void testSubtraction() {
Compiler compiler = new Compiler();
Node n = compiler.parseTestCode("x - -4");
assertEquals(0, compiler.getErrorCount());
assertEquals(
"x- -4",
printNode(n));
}
public void testFunctionWithCall() {
assertPrint(
"var user = new function() {"
+ "alert(\"foo\")}",
"var user=new function(){"
+ "alert(\"foo\")}");
assertPrint(
"var user = new function() {"
+ "this.name = \"foo\";"
+ "this.local = function(){alert(this.name)};}",
"var user=new function(){"
+ "this.name=\"foo\";"
+ "this.local=function(){alert(this.name)}}");
}
public void testLineLength() {
// list
assertLineLength("var aba,bcb,cdc",
"var aba,bcb," +
"\ncdc");
// operators, and two breaks
assertLineLength(
"\"foo\"+\"bar,baz,bomb\"+\"whee\"+\";long-string\"\n+\"aaa\"",
"\"foo\"+\"bar,baz,bomb\"+" +
"\n\"whee\"+\";long-string\"+" +
"\n\"aaa\"");
// assignment
assertLineLength("var abazaba=1234",
"var abazaba=" +
"\n1234");
// statements
assertLineLength("var abab=1;var bab=2",
"var abab=1;" +
"\nvar bab=2");
// don't break regexes
assertLineLength("var a=/some[reg](ex),with.*we?rd|chars/i;var b=a",
"var a=/some[reg](ex),with.*we?rd|chars/i;" +
"\nvar b=a");
// don't break strings
assertLineLength("var a=\"foo,{bar};baz\";var b=a",
"var a=\"foo,{bar};baz\";" +
"\nvar b=a");
// don't break before post inc/dec
assertLineLength("var a=\"a\";a++;var b=\"bbb\";",
"var a=\"a\";a++;\n" +
"var b=\"bbb\"");
}
private void assertLineLength(String js, String expected) {
assertEquals(expected,
parsePrint(js, false, true, 10));
}
public void testParsePrintParse() {
testReparse("3;");
testReparse("var a = b;");
testReparse("var x, y, z;");
testReparse("try { foo() } catch(e) { bar() }");
testReparse("try { foo() } catch(e) { bar() } finally { stuff() }");
testReparse("try { foo() } finally { stuff() }");
testReparse("throw 'me'");
testReparse("function foo(a) { return a + 4; }");
testReparse("function foo() { return; }");
testReparse("var a = function(a, b) { foo(); return a + b; }");
testReparse("b = [3, 4, 'paul', \"Buchhe it\",,5];");
testReparse("v = (5, 6, 7, 8)");
testReparse("d = 34.0; x = 0; y = .3; z = -22");
testReparse("d = -x; t = !x + ~y;");
testReparse("'hi'; /* just a test */ stuff(a,b) \n" +
" foo(); // and another \n" +
" bar();");
testReparse("a = b++ + ++c; a = b++-++c; a = - --b; a = - ++b;");
testReparse("a++; b= a++; b = ++a; b = a--; b = --a; a+=2; b-=5");
testReparse("a = (2 + 3) * 4;");
testReparse("a = 1 + (2 + 3) + 4;");
testReparse("x = a ? b : c; x = a ? (b,3,5) : (foo(),bar());");
testReparse("a = b | c || d ^ e " +
"&& f & !g != h << i <= j < k >>> l > m * n % !o");
testReparse("a == b; a != b; a === b; a == b == a;" +
" (a == b) == a; a == (b == a);");
testReparse("if (a > b) a = b; if (b < 3) a = 3; else c = 4;");
testReparse("if (a == b) { a++; } if (a == 0) { a++; } else { a --; }");
testReparse("for (var i in a) b += i;");
testReparse("for (var i = 0; i < 10; i++){ b /= 2;" +
" if (b == 2)break;else continue;}");
testReparse("for (x = 0; x < 10; x++) a /= 2;");
testReparse("for (;;) a++;");
testReparse("while(true) { blah(); }while(true) blah();");
testReparse("do stuff(); while(a>b);");
testReparse("[0, null, , true, false, this];");
testReparse("s.replace(/absc/, 'X').replace(/ab/gi, 'Y');");
testReparse("new Foo; new Bar(a, b,c);");
testReparse("with(foo()) { x = z; y = t; } with(bar()) a = z;");
testReparse("delete foo['bar']; delete foo;");
testReparse("var x = { 'a':'paul', 1:'3', 2:(3,4) };");
testReparse("switch(a) { case 2: case 3: stuff(); break;" +
"case 4: morestuff(); break; default: done();}");
testReparse("x = foo['bar'] + foo['my stuff'] + foo[bar] + f.stuff;");
testReparse("a.v = b.v; x['foo'] = y['zoo'];");
testReparse("'test' in x; 3 in x; a in x;");
testReparse("'foo\"bar' + \"foo'c\" + 'stuff\\n and \\\\more'");
testReparse("x.__proto__;");
}
private void testReparse(String code) {
Compiler compiler = new Compiler();
Node parse1 = parse(code);
Node parse2 = parse(new CodePrinter.Builder(parse1).build());
String explanation = parse1.checkTreeEquals(parse2);
assertNull("\nExpected: " + compiler.toSource(parse1) +
"\nResult: " + compiler.toSource(parse2) +
"\n" + explanation, explanation);
}
public void testDoLoopIECompatiblity() {
// Do loops within IFs cause syntax errors in IE6 and IE7.
assertPrint("function f(){if(e1){do foo();while(e2)}else foo()}",
"function f(){if(e1){do foo();while(e2)}else foo()}");
assertPrint("function f(){if(e1)do foo();while(e2)else foo()}",
"function f(){if(e1){do foo();while(e2)}else foo()}");
assertPrint("if(x){do{foo()}while(y)}else bar()",
"if(x){do foo();while(y)}else bar()");
assertPrint("if(x)do{foo()}while(y);else bar()",
"if(x){do foo();while(y)}else bar()");
assertPrint("if(x){do{foo()}while(y)}",
"if(x){do foo();while(y)}");
assertPrint("if(x)do{foo()}while(y);",
"if(x){do foo();while(y)}");
assertPrint("if(x)A:do{foo()}while(y);",
"if(x){A:do foo();while(y)}");
assertPrint("var i = 0;a: do{b: do{i++;break b;} while(0);} while(0);",
"var i=0;a:do{b:do{i++;break b}while(0)}while(0)");
}
public void testFunctionSafariCompatiblity() {
// Functions within IFs cause syntax errors on Safari.
assertPrint("function f(){if(e1){function goo(){return true}}else foo()}",
"function f(){if(e1){function goo(){return true}}else foo()}");
assertPrint("function f(){if(e1)function goo(){return true}else foo()}",
"function f(){if(e1){function goo(){return true}}else foo()}");
assertPrint("if(e1){function goo(){return true}}",
"if(e1){function goo(){return true}}");
assertPrint("if(e1)function goo(){return true}",
"if(e1){function goo(){return true}}");
assertPrint("if(e1)A:function goo(){return true}",
"if(e1){A:function goo(){return true}}");
}
public void testExponents() {
assertPrintNumber("1", 1);
assertPrintNumber("10", 10);
assertPrintNumber("100", 100);
assertPrintNumber("1E3", 1000);
assertPrintNumber("1E4", 10000);
assertPrintNumber("1E5", 100000);
assertPrintNumber("-1", -1);
assertPrintNumber("-10", -10);
assertPrintNumber("-100", -100);
assertPrintNumber("-1E3", -1000);
assertPrintNumber("-12341234E4", -123412340000L);
assertPrintNumber("1E18", 1000000000000000000L);
assertPrintNumber("1E5", 100000.0);
assertPrintNumber("100000.1", 100000.1);
assertPrintNumber("1E-6", 0.000001);
assertPrintNumber("-0x38d7ea4c68001", -0x38d7ea4c68001L);
assertPrintNumber("0x38d7ea4c68001", 0x38d7ea4c68001L);
}
// Make sure to test as both a String and a Node, because
// negative numbers do not parse consistently from strings.
private void assertPrintNumber(String expected, double number) {
assertPrint(String.valueOf(number), expected);
assertPrintNode(expected, Node.newNumber(number));
}
private void assertPrintNumber(String expected, int number) {
assertPrint(String.valueOf(number), expected);
assertPrintNode(expected, Node.newNumber(number));
}
public void testDirectEval() {
assertPrint("eval('1');", "eval(\"1\")");
}
public void testIndirectEval() {
Node n = parse("eval('1');");
assertPrintNode("eval(\"1\")", n);
n.getFirstChild().getFirstChild().getFirstChild().putBooleanProp(
Node.DIRECT_EVAL, false);
assertPrintNode("(0,eval)(\"1\")", n);
}
public void testFreeCall1() {
assertPrint("foo(a);", "foo(a)");
assertPrint("x.foo(a);", "x.foo(a)");
}
public void testFreeCall2() {
Node n = parse("foo(a);");
assertPrintNode("foo(a)", n);
Node call = n.getFirstChild().getFirstChild();
assertTrue(call.isCall());
call.putBooleanProp(Node.FREE_CALL, true);
assertPrintNode("foo(a)", n);
}
public void testFreeCall3() {
Node n = parse("x.foo(a);");
assertPrintNode("x.foo(a)", n);
Node call = n.getFirstChild().getFirstChild();
assertTrue(call.isCall());
call.putBooleanProp(Node.FREE_CALL, true);
assertPrintNode("(0,x.foo)(a)", n);
}
public void testPrintScript() {
// Verify that SCRIPT nodes not marked as synthetic are printed as
// blocks.
Node ast = new Node(Token.SCRIPT,
new Node(Token.EXPR_RESULT, Node.newString("f")),
new Node(Token.EXPR_RESULT, Node.newString("g")));
String result = new CodePrinter.Builder(ast).setPrettyPrint(true).build();
assertEquals("\"f\";\n\"g\";\n", result);
}
public void testObjectLit() {
assertPrint("({x:1})", "({x:1})");
assertPrint("var x=({x:1})", "var x={x:1}");
assertPrint("var x={'x':1}", "var x={\"x\":1}");
assertPrint("var x={1:1}", "var x={1:1}");
assertPrint("({},42)+0", "({},42)+0");
}
public void testObjectLit2() {
assertPrint("var x={1:1}", "var x={1:1}");
assertPrint("var x={'1':1}", "var x={1:1}");
assertPrint("var x={'1.0':1}", "var x={\"1.0\":1}");
assertPrint("var x={1.5:1}", "var x={\"1.5\":1}");
}
public void testObjectLit3() {
assertPrint("var x={3E9:1}",
"var x={3E9:1}");
assertPrint("var x={'3000000000':1}", // More than 31 bits
"var x={3E9:1}");
assertPrint("var x={'3000000001':1}",
"var x={3000000001:1}");
assertPrint("var x={'6000000001':1}", // More than 32 bits
"var x={6000000001:1}");
assertPrint("var x={\"12345678901234567\":1}", // More than 53 bits
"var x={\"12345678901234567\":1}");
}
public void testObjectLit4() {
// More than 128 bits.
assertPrint(
"var x={\"123456789012345671234567890123456712345678901234567\":1}",
"var x={\"123456789012345671234567890123456712345678901234567\":1}");
}
public void testGetter() {
assertPrint("var x = {}", "var x={}");
assertPrint("var x = {get a() {return 1}}", "var x={get a(){return 1}}");
assertPrint(
"var x = {get a() {}, get b(){}}",
"var x={get a(){},get b(){}}");
assertPrint(
"var x = {get 'a'() {return 1}}",
"var x={get \"a\"(){return 1}}");
assertPrint(
"var x = {get 1() {return 1}}",
"var x={get 1(){return 1}}");
assertPrint(
"var x = {get \"()\"() {return 1}}",
"var x={get \"()\"(){return 1}}");
languageMode = LanguageMode.ECMASCRIPT5;
assertPrintSame("var x={get function(){return 1}}");
// Getters and setters and not supported in ES3 but if someone sets the
// the ES3 output mode on an AST containing them we still produce them.
languageMode = LanguageMode.ECMASCRIPT3;
assertPrintSame("var x={get function(){return 1}}");
}
public void testSetter() {
assertPrint("var x = {}", "var x={}");
assertPrint(
"var x = {set a(y) {return 1}}",
"var x={set a(y){return 1}}");
assertPrint(
"var x = {get 'a'() {return 1}}",
"var x={get \"a\"(){return 1}}");
assertPrint(
"var x = {set 1(y) {return 1}}",
"var x={set 1(y){return 1}}");
assertPrint(
"var x = {set \"(x)\"(y) {return 1}}",
"var x={set \"(x)\"(y){return 1}}");
languageMode = LanguageMode.ECMASCRIPT5;
assertPrintSame("var x={set function(x){}}");
// Getters and setters and not supported in ES3 but if someone sets the
// the ES3 output mode on an AST containing them we still produce them.
languageMode = LanguageMode.ECMASCRIPT3;
assertPrintSame("var x={set function(x){}}");
}
public void testNegCollapse() {
// Collapse the negative symbol on numbers at generation time,
// to match the Rhino behavior.
assertPrint("var x = - - 2;", "var x=2");
assertPrint("var x = - (2);", "var x=-2");
}
public void testStrict() {
String result = parsePrint("var x", false, false, 0, false, true);
assertEquals("'use strict';var x", result);
}
public void testArrayLiteral() {
assertPrint("var x = [,];","var x=[,]");
assertPrint("var x = [,,];","var x=[,,]");
assertPrint("var x = [,s,,];","var x=[,s,,]");
assertPrint("var x = [,s];","var x=[,s]");
assertPrint("var x = [s,];","var x=[s]");
}
public void testZero() {
assertPrint("var x ='\\0';", "var x=\"\\x00\"");
assertPrint("var x ='\\x00';", "var x=\"\\x00\"");
assertPrint("var x ='\\u0000';", "var x=\"\\x00\"");
assertPrint("var x ='\\u00003';", "var x=\"\\x003\"");
}
public void testUnicode() {
assertPrint("var x ='\\x0f';", "var x=\"\\u000f\"");
assertPrint("var x ='\\x68';", "var x=\"h\"");
assertPrint("var x ='\\x7f';", "var x=\"\\u007f\"");
}
public void testUnicodeKeyword() {
// keyword "if"
assertPrint("var \\u0069\\u0066 = 1;", "var i\\u0066=1");
// keyword "var"
assertPrint("var v\\u0061\\u0072 = 1;", "var va\\u0072=1");
// all are keyword "while"
assertPrint("var w\\u0068\\u0069\\u006C\\u0065 = 1;"
+ "\\u0077\\u0068il\\u0065 = 2;"
+ "\\u0077h\\u0069le = 3;",
"var whil\\u0065=1;whil\\u0065=2;whil\\u0065=3");
}
public void testNumericKeys() {
assertPrint("var x = {010: 1};", "var x={8:1}");
assertPrint("var x = {'010': 1};", "var x={\"010\":1}");
assertPrint("var x = {0x10: 1};", "var x={16:1}");
assertPrint("var x = {'0x10': 1};", "var x={\"0x10\":1}");
// I was surprised at this result too.
assertPrint("var x = {.2: 1};", "var x={\"0.2\":1}");
assertPrint("var x = {'.2': 1};", "var x={\".2\":1}");
assertPrint("var x = {0.2: 1};", "var x={\"0.2\":1}");
assertPrint("var x = {'0.2': 1};", "var x={\"0.2\":1}");
}
public void testIssue582() {
assertPrint("var x = -0.0;", "var x=-0");
}
public void testIssue942() {
assertPrint("var x = {0: 1};", "var x={0:1}");
}
public void testIssue601() {
assertPrint("'\\v' == 'v'", "\"\\v\"==\"v\"");
assertPrint("'\\u000B' == '\\v'", "\"\\x0B\"==\"\\v\"");
assertPrint("'\\x0B' == '\\v'", "\"\\x0B\"==\"\\v\"");
}
public void testIssue620() {
assertPrint("alert(/ / / / /);", "alert(/ // / /)");
assertPrint("alert(/ // / /);", "alert(/ // / /)");
}
public void testIssue5746867() {
assertPrint("var a = { '$\\\\' : 5 };", "var a={\"$\\\\\":5}");
}
public void testCommaSpacing() {
assertPrint("var a = (b = 5, c = 5);",
"var a=(b=5,c=5)");
assertPrettyPrint("var a = (b = 5, c = 5);",
"var a = (b = 5, c = 5);\n");
}
public void testManyCommas() {
int numCommas = 10000;
List<String> numbers = Lists.newArrayList("0", "1");
Node current = new Node(Token.COMMA, Node.newNumber(0), Node.newNumber(1));
for (int i = 2; i < numCommas; i++) {
current = new Node(Token.COMMA, current);
// 1000 is printed as 1E3, and screws up our test.
int num = i % 1000;
numbers.add(String.valueOf(num));
current.addChildToBack(Node.newNumber(num));
}
String expected = Joiner.on(",").join(numbers);
String actual = printNode(current).replace("\n", "");
assertEquals(expected, actual);
}
public void testManyAdds() {
int numAdds = 10000;
List<String> numbers = Lists.newArrayList("0", "1");
Node current = new Node(Token.ADD, Node.newNumber(0), Node.newNumber(1));
for (int i = 2; i < numAdds; i++) {
current = new Node(Token.ADD, current);
// 1000 is printed as 1E3, and screws up our test.
int num = i % 1000;
numbers.add(String.valueOf(num));
current.addChildToBack(Node.newNumber(num));
}
String expected = Joiner.on("+").join(numbers);
String actual = printNode(current).replace("\n", "");
assertEquals(expected, actual);
}
public void testMinusNegativeZero() {
// Negative zero is weird, because we have to be able to distinguish
// it from positive zero (there are some subtle differences in behavior).
assertPrint("x- -0", "x- -0");
}
public void testStringEscapeSequences() {
// From the SingleEscapeCharacter grammar production.
assertPrintSame("var x=\"\\b\"");
assertPrintSame("var x=\"\\f\"");
assertPrintSame("var x=\"\\n\"");
assertPrintSame("var x=\"\\r\"");
assertPrintSame("var x=\"\\t\"");
assertPrintSame("var x=\"\\v\"");
assertPrint("var x=\"\\\"\"", "var x='\"'");
assertPrint("var x=\"\\\'\"", "var x=\"'\"");
// From the LineTerminator grammar
assertPrint("var x=\"\\u000A\"", "var x=\"\\n\"");
assertPrint("var x=\"\\u000D\"", "var x=\"\\r\"");
assertPrintSame("var x=\"\\u2028\"");
assertPrintSame("var x=\"\\u2029\"");
// Now with regular expressions.
assertPrintSame("var x=/\\b/");
assertPrintSame("var x=/\\f/");
assertPrintSame("var x=/\\n/");
assertPrintSame("var x=/\\r/");
assertPrintSame("var x=/\\t/");
assertPrintSame("var x=/\\v/");
assertPrintSame("var x=/\\u000A/");
assertPrintSame("var x=/\\u000D/");
assertPrintSame("var x=/\\u2028/");
assertPrintSame("var x=/\\u2029/");
}
public void testKeywordProperties1() {
languageMode = LanguageMode.ECMASCRIPT5;
assertPrintSame("x.foo=2");
assertPrintSame("x.function=2");
languageMode = LanguageMode.ECMASCRIPT3;
assertPrintSame("x.foo=2");
assertPrint("x.function=2", "x[\"function\"]=2");
}
public void testKeywordProperties2() {
languageMode = LanguageMode.ECMASCRIPT5;
assertPrintSame("x={foo:2}");
assertPrintSame("x={function:2}");
languageMode = LanguageMode.ECMASCRIPT3;
assertPrintSame("x={foo:2}");
assertPrint("x={function:2}", "x={\"function\":2}");
}
} |
||
public void testNestedUnwrappedLists180() throws Exception
{
/*
Records recs = new Records();
recs.records.add(new Record());
recs.records.add(new Record());
recs.records.add(new Record());
recs.records.get(0).fields.add(new Field("a"));
recs.records.get(2).fields.add(new Field("b"));
String xml = MAPPER.writerWithDefaultPrettyPrinter().writeValueAsString(recs);
*/
String xml =
"<Records>\n"
// Important: it's the empty CDATA here that causes breakage -- empty element alone would be fine
//+"<records>\n</records>\n"
+"<records></records>\n"
+" <records>\n"
+" <fields name='b'/>\n"
+" </records>\n"
+"</Records>\n"
;
//System.out.println("XML: "+xml);
Records result = MAPPER.readValue(xml, Records.class);
assertNotNull(result.records);
assertEquals(2, result.records.size());
assertNotNull(result.records.get(1));
assertEquals(1, result.records.get(1).fields.size());
assertEquals("b", result.records.get(1).fields.get(0).name);
// also, first one ought not be null should it? Ideally not...
assertNotNull(result.records.get(0));
} | com.fasterxml.jackson.dataformat.xml.lists.NestedUnwrappedLists180Test::testNestedUnwrappedLists180 | src/test/java/com/fasterxml/jackson/dataformat/xml/lists/NestedUnwrappedLists180Test.java | 72 | src/test/java/com/fasterxml/jackson/dataformat/xml/lists/NestedUnwrappedLists180Test.java | testNestedUnwrappedLists180 | package com.fasterxml.jackson.dataformat.xml.lists;
import java.util.*;
import com.fasterxml.jackson.dataformat.xml.XmlMapper;
import com.fasterxml.jackson.dataformat.xml.XmlTestBase;
import com.fasterxml.jackson.dataformat.xml.annotation.JacksonXmlElementWrapper;
import com.fasterxml.jackson.dataformat.xml.annotation.JacksonXmlProperty;
public class NestedUnwrappedLists180Test extends XmlTestBase
{
static class Records {
@JacksonXmlElementWrapper(useWrapping=false)
public List<Record> records = new ArrayList<Record>();
}
static class Record {
@JacksonXmlElementWrapper(useWrapping=false)
public List<Field> fields = new ArrayList<Field>();
}
static class Field {
@JacksonXmlProperty(isAttribute=true)
public String name;
protected Field() { }
public Field(String n) { name = n; }
}
/*
/**********************************************************************
/* Unit tests
/**********************************************************************
*/
private final XmlMapper MAPPER = new XmlMapper();
public void testNestedUnwrappedLists180() throws Exception
{
/*
Records recs = new Records();
recs.records.add(new Record());
recs.records.add(new Record());
recs.records.add(new Record());
recs.records.get(0).fields.add(new Field("a"));
recs.records.get(2).fields.add(new Field("b"));
String xml = MAPPER.writerWithDefaultPrettyPrinter().writeValueAsString(recs);
*/
String xml =
"<Records>\n"
// Important: it's the empty CDATA here that causes breakage -- empty element alone would be fine
//+"<records>\n</records>\n"
+"<records></records>\n"
+" <records>\n"
+" <fields name='b'/>\n"
+" </records>\n"
+"</Records>\n"
;
//System.out.println("XML: "+xml);
Records result = MAPPER.readValue(xml, Records.class);
assertNotNull(result.records);
assertEquals(2, result.records.size());
assertNotNull(result.records.get(1));
assertEquals(1, result.records.get(1).fields.size());
assertEquals("b", result.records.get(1).fields.get(0).name);
// also, first one ought not be null should it? Ideally not...
assertNotNull(result.records.get(0));
}
} | // You are a professional Java test case writer, please create a test case named `testNestedUnwrappedLists180` for the issue `JacksonXml-180`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonXml-180
//
// ## Issue-Title:
// Problem with deserialization of nested non-wrapped lists, with empty inner list
//
// ## Issue-Description:
// Looks like there is a problem, wherein nested structures like say:
//
//
// * Definition POJO, with `records`, unwrapped List with `Record`
// * `Record` POJO having property `fields`, another unwrapped list of `Field` POJOs
//
//
// and case where inner `List` happens to be empty/missing, cause incorrectly "split" parts of outermost `List`s (here for property `records`).
//
//
// I will come up with a full reproduction later on, but observed this in the wild, and I think it occurs with latest 2.7.0-rc code, as well as `2.6.4-1`, so is not just something that has been fixed with a later version.
//
//
//
//
public void testNestedUnwrappedLists180() throws Exception {
| 72 | 1 | 37 | src/test/java/com/fasterxml/jackson/dataformat/xml/lists/NestedUnwrappedLists180Test.java | src/test/java | ```markdown
## Issue-ID: JacksonXml-180
## Issue-Title:
Problem with deserialization of nested non-wrapped lists, with empty inner list
## Issue-Description:
Looks like there is a problem, wherein nested structures like say:
* Definition POJO, with `records`, unwrapped List with `Record`
* `Record` POJO having property `fields`, another unwrapped list of `Field` POJOs
and case where inner `List` happens to be empty/missing, cause incorrectly "split" parts of outermost `List`s (here for property `records`).
I will come up with a full reproduction later on, but observed this in the wild, and I think it occurs with latest 2.7.0-rc code, as well as `2.6.4-1`, so is not just something that has been fixed with a later version.
```
You are a professional Java test case writer, please create a test case named `testNestedUnwrappedLists180` for the issue `JacksonXml-180`, utilizing the provided issue report information and the following function signature.
```java
public void testNestedUnwrappedLists180() throws Exception {
```
| 37 | [
"com.fasterxml.jackson.dataformat.xml.deser.FromXmlParser"
] | a57e5cc4e6d83a176e3ad4e4fb97da33881889324dcb64840681f519b508f27c | public void testNestedUnwrappedLists180() throws Exception
| // You are a professional Java test case writer, please create a test case named `testNestedUnwrappedLists180` for the issue `JacksonXml-180`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: JacksonXml-180
//
// ## Issue-Title:
// Problem with deserialization of nested non-wrapped lists, with empty inner list
//
// ## Issue-Description:
// Looks like there is a problem, wherein nested structures like say:
//
//
// * Definition POJO, with `records`, unwrapped List with `Record`
// * `Record` POJO having property `fields`, another unwrapped list of `Field` POJOs
//
//
// and case where inner `List` happens to be empty/missing, cause incorrectly "split" parts of outermost `List`s (here for property `records`).
//
//
// I will come up with a full reproduction later on, but observed this in the wild, and I think it occurs with latest 2.7.0-rc code, as well as `2.6.4-1`, so is not just something that has been fixed with a later version.
//
//
//
//
| JacksonXml | package com.fasterxml.jackson.dataformat.xml.lists;
import java.util.*;
import com.fasterxml.jackson.dataformat.xml.XmlMapper;
import com.fasterxml.jackson.dataformat.xml.XmlTestBase;
import com.fasterxml.jackson.dataformat.xml.annotation.JacksonXmlElementWrapper;
import com.fasterxml.jackson.dataformat.xml.annotation.JacksonXmlProperty;
public class NestedUnwrappedLists180Test extends XmlTestBase
{
static class Records {
@JacksonXmlElementWrapper(useWrapping=false)
public List<Record> records = new ArrayList<Record>();
}
static class Record {
@JacksonXmlElementWrapper(useWrapping=false)
public List<Field> fields = new ArrayList<Field>();
}
static class Field {
@JacksonXmlProperty(isAttribute=true)
public String name;
protected Field() { }
public Field(String n) { name = n; }
}
/*
/**********************************************************************
/* Unit tests
/**********************************************************************
*/
private final XmlMapper MAPPER = new XmlMapper();
public void testNestedUnwrappedLists180() throws Exception
{
/*
Records recs = new Records();
recs.records.add(new Record());
recs.records.add(new Record());
recs.records.add(new Record());
recs.records.get(0).fields.add(new Field("a"));
recs.records.get(2).fields.add(new Field("b"));
String xml = MAPPER.writerWithDefaultPrettyPrinter().writeValueAsString(recs);
*/
String xml =
"<Records>\n"
// Important: it's the empty CDATA here that causes breakage -- empty element alone would be fine
//+"<records>\n</records>\n"
+"<records></records>\n"
+" <records>\n"
+" <fields name='b'/>\n"
+" </records>\n"
+"</Records>\n"
;
//System.out.println("XML: "+xml);
Records result = MAPPER.readValue(xml, Records.class);
assertNotNull(result.records);
assertEquals(2, result.records.size());
assertNotNull(result.records.get(1));
assertEquals(1, result.records.get(1).fields.size());
assertEquals("b", result.records.get(1).fields.get(0).name);
// also, first one ought not be null should it? Ideally not...
assertNotNull(result.records.get(0));
}
} |
||
public void test_toBoolean_String() {
assertEquals(false, BooleanUtils.toBoolean((String) null));
assertEquals(false, BooleanUtils.toBoolean(""));
assertEquals(false, BooleanUtils.toBoolean("off"));
assertEquals(false, BooleanUtils.toBoolean("oof"));
assertEquals(false, BooleanUtils.toBoolean("yep"));
assertEquals(false, BooleanUtils.toBoolean("trux"));
assertEquals(false, BooleanUtils.toBoolean("false"));
assertEquals(false, BooleanUtils.toBoolean("a"));
assertEquals(true, BooleanUtils.toBoolean("true")); // interned handled differently
assertEquals(true, BooleanUtils.toBoolean(new StringBuffer("tr").append("ue").toString()));
assertEquals(true, BooleanUtils.toBoolean("truE"));
assertEquals(true, BooleanUtils.toBoolean("trUe"));
assertEquals(true, BooleanUtils.toBoolean("trUE"));
assertEquals(true, BooleanUtils.toBoolean("tRue"));
assertEquals(true, BooleanUtils.toBoolean("tRuE"));
assertEquals(true, BooleanUtils.toBoolean("tRUe"));
assertEquals(true, BooleanUtils.toBoolean("tRUE"));
assertEquals(true, BooleanUtils.toBoolean("TRUE"));
assertEquals(true, BooleanUtils.toBoolean("TRUe"));
assertEquals(true, BooleanUtils.toBoolean("TRuE"));
assertEquals(true, BooleanUtils.toBoolean("TRue"));
assertEquals(true, BooleanUtils.toBoolean("TrUE"));
assertEquals(true, BooleanUtils.toBoolean("TrUe"));
assertEquals(true, BooleanUtils.toBoolean("TruE"));
assertEquals(true, BooleanUtils.toBoolean("True"));
assertEquals(true, BooleanUtils.toBoolean("on"));
assertEquals(true, BooleanUtils.toBoolean("oN"));
assertEquals(true, BooleanUtils.toBoolean("On"));
assertEquals(true, BooleanUtils.toBoolean("ON"));
assertEquals(true, BooleanUtils.toBoolean("yes"));
assertEquals(true, BooleanUtils.toBoolean("yeS"));
assertEquals(true, BooleanUtils.toBoolean("yEs"));
assertEquals(true, BooleanUtils.toBoolean("yES"));
assertEquals(true, BooleanUtils.toBoolean("Yes"));
assertEquals(true, BooleanUtils.toBoolean("YeS"));
assertEquals(true, BooleanUtils.toBoolean("YEs"));
assertEquals(true, BooleanUtils.toBoolean("YES"));
assertEquals(false, BooleanUtils.toBoolean("yes?"));
assertEquals(false, BooleanUtils.toBoolean("tru"));
} | org.apache.commons.lang.BooleanUtilsTest::test_toBoolean_String | src/test/org/apache/commons/lang/BooleanUtilsTest.java | 334 | src/test/org/apache/commons/lang/BooleanUtilsTest.java | test_toBoolean_String | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.lang;
import java.lang.reflect.Constructor;
import java.lang.reflect.Modifier;
import junit.framework.Test;
import junit.framework.TestCase;
import junit.framework.TestSuite;
import junit.textui.TestRunner;
/**
* Unit tests {@link org.apache.commons.lang.BooleanUtils}.
*
* @author Stephen Colebourne
* @author Matthew Hawthorne
* @version $Id$
*/
public class BooleanUtilsTest extends TestCase {
public BooleanUtilsTest(String name) {
super(name);
}
public static void main(String[] args) {
TestRunner.run(suite());
}
public static Test suite() {
TestSuite suite = new TestSuite(BooleanUtilsTest.class);
suite.setName("BooleanUtils Tests");
return suite;
}
protected void setUp() throws Exception {
super.setUp();
}
protected void tearDown() throws Exception {
super.tearDown();
}
//-----------------------------------------------------------------------
public void testConstructor() {
assertNotNull(new BooleanUtils());
Constructor[] cons = BooleanUtils.class.getDeclaredConstructors();
assertEquals(1, cons.length);
assertEquals(true, Modifier.isPublic(cons[0].getModifiers()));
assertEquals(true, Modifier.isPublic(BooleanUtils.class.getModifiers()));
assertEquals(false, Modifier.isFinal(BooleanUtils.class.getModifiers()));
}
//-----------------------------------------------------------------------
public void test_negate_Boolean() {
assertSame(null, BooleanUtils.negate(null));
assertSame(Boolean.TRUE, BooleanUtils.negate(Boolean.FALSE));
assertSame(Boolean.FALSE, BooleanUtils.negate(Boolean.TRUE));
}
//-----------------------------------------------------------------------
public void test_isTrue_Boolean() {
assertEquals(true, BooleanUtils.isTrue(Boolean.TRUE));
assertEquals(false, BooleanUtils.isTrue(Boolean.FALSE));
assertEquals(false, BooleanUtils.isTrue((Boolean) null));
}
public void test_isNotTrue_Boolean() {
assertEquals(false, BooleanUtils.isNotTrue(Boolean.TRUE));
assertEquals(true, BooleanUtils.isNotTrue(Boolean.FALSE));
assertEquals(true, BooleanUtils.isNotTrue((Boolean) null));
}
//-----------------------------------------------------------------------
public void test_isFalse_Boolean() {
assertEquals(false, BooleanUtils.isFalse(Boolean.TRUE));
assertEquals(true, BooleanUtils.isFalse(Boolean.FALSE));
assertEquals(false, BooleanUtils.isFalse((Boolean) null));
}
public void test_isNotFalse_Boolean() {
assertEquals(true, BooleanUtils.isNotFalse(Boolean.TRUE));
assertEquals(false, BooleanUtils.isNotFalse(Boolean.FALSE));
assertEquals(true, BooleanUtils.isNotFalse((Boolean) null));
}
//-----------------------------------------------------------------------
public void test_toBooleanObject_boolean() {
assertSame(Boolean.TRUE, BooleanUtils.toBooleanObject(true));
assertSame(Boolean.FALSE, BooleanUtils.toBooleanObject(false));
}
public void test_toBoolean_Boolean() {
assertEquals(true, BooleanUtils.toBoolean(Boolean.TRUE));
assertEquals(false, BooleanUtils.toBoolean(Boolean.FALSE));
assertEquals(false, BooleanUtils.toBoolean((Boolean) null));
}
public void test_toBooleanDefaultIfNull_Boolean_boolean() {
assertEquals(true, BooleanUtils.toBooleanDefaultIfNull(Boolean.TRUE, true));
assertEquals(true, BooleanUtils.toBooleanDefaultIfNull(Boolean.TRUE, false));
assertEquals(false, BooleanUtils.toBooleanDefaultIfNull(Boolean.FALSE, true));
assertEquals(false, BooleanUtils.toBooleanDefaultIfNull(Boolean.FALSE, false));
assertEquals(true, BooleanUtils.toBooleanDefaultIfNull((Boolean) null, true));
assertEquals(false, BooleanUtils.toBooleanDefaultIfNull((Boolean) null, false));
}
//-----------------------------------------------------------------------
//-----------------------------------------------------------------------
public void test_toBoolean_int() {
assertEquals(true, BooleanUtils.toBoolean(1));
assertEquals(true, BooleanUtils.toBoolean(-1));
assertEquals(false, BooleanUtils.toBoolean(0));
}
public void test_toBooleanObject_int() {
assertEquals(Boolean.TRUE, BooleanUtils.toBooleanObject(1));
assertEquals(Boolean.TRUE, BooleanUtils.toBooleanObject(-1));
assertEquals(Boolean.FALSE, BooleanUtils.toBooleanObject(0));
}
public void test_toBooleanObject_Integer() {
assertEquals(Boolean.TRUE, BooleanUtils.toBooleanObject(new Integer(1)));
assertEquals(Boolean.TRUE, BooleanUtils.toBooleanObject(new Integer(-1)));
assertEquals(Boolean.FALSE, BooleanUtils.toBooleanObject(new Integer(0)));
assertEquals(null, BooleanUtils.toBooleanObject((Integer) null));
}
//-----------------------------------------------------------------------
public void test_toBoolean_int_int_int() {
assertEquals(true, BooleanUtils.toBoolean(6, 6, 7));
assertEquals(false, BooleanUtils.toBoolean(7, 6, 7));
try {
BooleanUtils.toBoolean(8, 6, 7);
fail();
} catch (IllegalArgumentException ex) {}
}
public void test_toBoolean_Integer_Integer_Integer() {
Integer six = new Integer(6);
Integer seven = new Integer(7);
assertEquals(true, BooleanUtils.toBoolean((Integer) null, null, seven));
assertEquals(false, BooleanUtils.toBoolean((Integer) null, six, null));
try {
BooleanUtils.toBoolean(null, six, seven);
fail();
} catch (IllegalArgumentException ex) {}
assertEquals(true, BooleanUtils.toBoolean(new Integer(6), six, seven));
assertEquals(false, BooleanUtils.toBoolean(new Integer(7), six, seven));
try {
BooleanUtils.toBoolean(new Integer(8), six, seven);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void test_toBooleanObject_int_int_int() {
assertEquals(Boolean.TRUE, BooleanUtils.toBooleanObject(6, 6, 7, 8));
assertEquals(Boolean.FALSE, BooleanUtils.toBooleanObject(7, 6, 7, 8));
assertEquals(null, BooleanUtils.toBooleanObject(8, 6, 7, 8));
try {
BooleanUtils.toBooleanObject(9, 6, 7, 8);
fail();
} catch (IllegalArgumentException ex) {}
}
public void test_toBooleanObject_Integer_Integer_Integer_Integer() {
Integer six = new Integer(6);
Integer seven = new Integer(7);
Integer eight = new Integer(8);
assertSame(Boolean.TRUE, BooleanUtils.toBooleanObject((Integer) null, null, seven, eight));
assertSame(Boolean.FALSE, BooleanUtils.toBooleanObject((Integer) null, six, null, eight));
assertSame(null, BooleanUtils.toBooleanObject((Integer) null, six, seven, null));
try {
BooleanUtils.toBooleanObject(null, six, seven, eight);
fail();
} catch (IllegalArgumentException ex) {}
assertEquals(Boolean.TRUE, BooleanUtils.toBooleanObject(new Integer(6), six, seven, eight));
assertEquals(Boolean.FALSE, BooleanUtils.toBooleanObject(new Integer(7), six, seven, eight));
assertEquals(null, BooleanUtils.toBooleanObject(new Integer(8), six, seven, eight));
try {
BooleanUtils.toBooleanObject(new Integer(9), six, seven, eight);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void test_toInteger_boolean() {
assertEquals(1, BooleanUtils.toInteger(true));
assertEquals(0, BooleanUtils.toInteger(false));
}
public void test_toIntegerObject_boolean() {
assertEquals(new Integer(1), BooleanUtils.toIntegerObject(true));
assertEquals(new Integer(0), BooleanUtils.toIntegerObject(false));
}
public void test_toIntegerObject_Boolean() {
assertEquals(new Integer(1), BooleanUtils.toIntegerObject(Boolean.TRUE));
assertEquals(new Integer(0), BooleanUtils.toIntegerObject(Boolean.FALSE));
assertEquals(null, BooleanUtils.toIntegerObject((Boolean) null));
}
//-----------------------------------------------------------------------
public void test_toInteger_boolean_int_int() {
assertEquals(6, BooleanUtils.toInteger(true, 6, 7));
assertEquals(7, BooleanUtils.toInteger(false, 6, 7));
}
public void test_toInteger_Boolean_int_int_int() {
assertEquals(6, BooleanUtils.toInteger(Boolean.TRUE, 6, 7, 8));
assertEquals(7, BooleanUtils.toInteger(Boolean.FALSE, 6, 7, 8));
assertEquals(8, BooleanUtils.toInteger(null, 6, 7, 8));
}
public void test_toIntegerObject_boolean_Integer_Integer() {
Integer six = new Integer(6);
Integer seven = new Integer(7);
assertEquals(six, BooleanUtils.toIntegerObject(true, six, seven));
assertEquals(seven, BooleanUtils.toIntegerObject(false, six, seven));
}
public void test_toIntegerObject_Boolean_Integer_Integer_Integer() {
Integer six = new Integer(6);
Integer seven = new Integer(7);
Integer eight = new Integer(8);
assertEquals(six, BooleanUtils.toIntegerObject(Boolean.TRUE, six, seven, eight));
assertEquals(seven, BooleanUtils.toIntegerObject(Boolean.FALSE, six, seven, eight));
assertEquals(eight, BooleanUtils.toIntegerObject((Boolean) null, six, seven, eight));
assertEquals(null, BooleanUtils.toIntegerObject((Boolean) null, six, seven, null));
}
//-----------------------------------------------------------------------
//-----------------------------------------------------------------------
public void test_toBooleanObject_String() {
assertEquals(null, BooleanUtils.toBooleanObject((String) null));
assertEquals(null, BooleanUtils.toBooleanObject(""));
assertEquals(Boolean.FALSE, BooleanUtils.toBooleanObject("false"));
assertEquals(Boolean.FALSE, BooleanUtils.toBooleanObject("no"));
assertEquals(Boolean.FALSE, BooleanUtils.toBooleanObject("off"));
assertEquals(Boolean.FALSE, BooleanUtils.toBooleanObject("FALSE"));
assertEquals(Boolean.FALSE, BooleanUtils.toBooleanObject("NO"));
assertEquals(Boolean.FALSE, BooleanUtils.toBooleanObject("OFF"));
assertEquals(null, BooleanUtils.toBooleanObject("oof"));
assertEquals(Boolean.TRUE, BooleanUtils.toBooleanObject("true"));
assertEquals(Boolean.TRUE, BooleanUtils.toBooleanObject("yes"));
assertEquals(Boolean.TRUE, BooleanUtils.toBooleanObject("on"));
assertEquals(Boolean.TRUE, BooleanUtils.toBooleanObject("TRUE"));
assertEquals(Boolean.TRUE, BooleanUtils.toBooleanObject("ON"));
assertEquals(Boolean.TRUE, BooleanUtils.toBooleanObject("YES"));
assertEquals(Boolean.TRUE, BooleanUtils.toBooleanObject("TruE"));
}
public void test_toBooleanObject_String_String_String_String() {
assertSame(Boolean.TRUE, BooleanUtils.toBooleanObject((String) null, null, "N", "U"));
assertSame(Boolean.FALSE, BooleanUtils.toBooleanObject((String) null, "Y", null, "U"));
assertSame(null, BooleanUtils.toBooleanObject((String) null, "Y", "N", null));
try {
BooleanUtils.toBooleanObject((String) null, "Y", "N", "U");
fail();
} catch (IllegalArgumentException ex) {}
assertEquals(Boolean.TRUE, BooleanUtils.toBooleanObject("Y", "Y", "N", "U"));
assertEquals(Boolean.FALSE, BooleanUtils.toBooleanObject("N", "Y", "N", "U"));
assertEquals(null, BooleanUtils.toBooleanObject("U", "Y", "N", "U"));
try {
BooleanUtils.toBooleanObject(null, "Y", "N", "U");
fail();
} catch (IllegalArgumentException ex) {}
try {
BooleanUtils.toBooleanObject("X", "Y", "N", "U");
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void test_toBoolean_String() {
assertEquals(false, BooleanUtils.toBoolean((String) null));
assertEquals(false, BooleanUtils.toBoolean(""));
assertEquals(false, BooleanUtils.toBoolean("off"));
assertEquals(false, BooleanUtils.toBoolean("oof"));
assertEquals(false, BooleanUtils.toBoolean("yep"));
assertEquals(false, BooleanUtils.toBoolean("trux"));
assertEquals(false, BooleanUtils.toBoolean("false"));
assertEquals(false, BooleanUtils.toBoolean("a"));
assertEquals(true, BooleanUtils.toBoolean("true")); // interned handled differently
assertEquals(true, BooleanUtils.toBoolean(new StringBuffer("tr").append("ue").toString()));
assertEquals(true, BooleanUtils.toBoolean("truE"));
assertEquals(true, BooleanUtils.toBoolean("trUe"));
assertEquals(true, BooleanUtils.toBoolean("trUE"));
assertEquals(true, BooleanUtils.toBoolean("tRue"));
assertEquals(true, BooleanUtils.toBoolean("tRuE"));
assertEquals(true, BooleanUtils.toBoolean("tRUe"));
assertEquals(true, BooleanUtils.toBoolean("tRUE"));
assertEquals(true, BooleanUtils.toBoolean("TRUE"));
assertEquals(true, BooleanUtils.toBoolean("TRUe"));
assertEquals(true, BooleanUtils.toBoolean("TRuE"));
assertEquals(true, BooleanUtils.toBoolean("TRue"));
assertEquals(true, BooleanUtils.toBoolean("TrUE"));
assertEquals(true, BooleanUtils.toBoolean("TrUe"));
assertEquals(true, BooleanUtils.toBoolean("TruE"));
assertEquals(true, BooleanUtils.toBoolean("True"));
assertEquals(true, BooleanUtils.toBoolean("on"));
assertEquals(true, BooleanUtils.toBoolean("oN"));
assertEquals(true, BooleanUtils.toBoolean("On"));
assertEquals(true, BooleanUtils.toBoolean("ON"));
assertEquals(true, BooleanUtils.toBoolean("yes"));
assertEquals(true, BooleanUtils.toBoolean("yeS"));
assertEquals(true, BooleanUtils.toBoolean("yEs"));
assertEquals(true, BooleanUtils.toBoolean("yES"));
assertEquals(true, BooleanUtils.toBoolean("Yes"));
assertEquals(true, BooleanUtils.toBoolean("YeS"));
assertEquals(true, BooleanUtils.toBoolean("YEs"));
assertEquals(true, BooleanUtils.toBoolean("YES"));
assertEquals(false, BooleanUtils.toBoolean("yes?"));
assertEquals(false, BooleanUtils.toBoolean("tru"));
}
public void test_toBoolean_String_String_String() {
assertEquals(true, BooleanUtils.toBoolean((String) null, null, "N"));
assertEquals(false, BooleanUtils.toBoolean((String) null, "Y", null));
try {
BooleanUtils.toBooleanObject((String) null, "Y", "N", "U");
fail();
} catch (IllegalArgumentException ex) {}
assertEquals(true, BooleanUtils.toBoolean("Y", "Y", "N"));
assertEquals(false, BooleanUtils.toBoolean("N", "Y", "N"));
try {
BooleanUtils.toBoolean(null, "Y", "N");
fail();
} catch (IllegalArgumentException ex) {}
try {
BooleanUtils.toBoolean("X", "Y", "N");
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void test_toStringTrueFalse_Boolean() {
assertEquals(null, BooleanUtils.toStringTrueFalse((Boolean) null));
assertEquals("true", BooleanUtils.toStringTrueFalse(Boolean.TRUE));
assertEquals("false", BooleanUtils.toStringTrueFalse(Boolean.FALSE));
}
public void test_toStringOnOff_Boolean() {
assertEquals(null, BooleanUtils.toStringOnOff((Boolean) null));
assertEquals("on", BooleanUtils.toStringOnOff(Boolean.TRUE));
assertEquals("off", BooleanUtils.toStringOnOff(Boolean.FALSE));
}
public void test_toStringYesNo_Boolean() {
assertEquals(null, BooleanUtils.toStringYesNo((Boolean) null));
assertEquals("yes", BooleanUtils.toStringYesNo(Boolean.TRUE));
assertEquals("no", BooleanUtils.toStringYesNo(Boolean.FALSE));
}
public void test_toString_Boolean_String_String_String() {
assertEquals("U", BooleanUtils.toString((Boolean) null, "Y", "N", "U"));
assertEquals("Y", BooleanUtils.toString(Boolean.TRUE, "Y", "N", "U"));
assertEquals("N", BooleanUtils.toString(Boolean.FALSE, "Y", "N", "U"));
}
//-----------------------------------------------------------------------
public void test_toStringTrueFalse_boolean() {
assertEquals("true", BooleanUtils.toStringTrueFalse(true));
assertEquals("false", BooleanUtils.toStringTrueFalse(false));
}
public void test_toStringOnOff_boolean() {
assertEquals("on", BooleanUtils.toStringOnOff(true));
assertEquals("off", BooleanUtils.toStringOnOff(false));
}
public void test_toStringYesNo_boolean() {
assertEquals("yes", BooleanUtils.toStringYesNo(true));
assertEquals("no", BooleanUtils.toStringYesNo(false));
}
public void test_toString_boolean_String_String_String() {
assertEquals("Y", BooleanUtils.toString(true, "Y", "N"));
assertEquals("N", BooleanUtils.toString(false, "Y", "N"));
}
// testXor
// -----------------------------------------------------------------------
public void testXor_primitive_nullInput() {
final boolean[] b = null;
try {
BooleanUtils.xor(b);
fail("Exception was not thrown for null input.");
} catch (IllegalArgumentException ex) {}
}
public void testXor_primitive_emptyInput() {
try {
BooleanUtils.xor(new boolean[] {});
fail("Exception was not thrown for empty input.");
} catch (IllegalArgumentException ex) {}
}
public void testXor_primitive_validInput_2items() {
assertTrue(
"True result for (true, true)",
! BooleanUtils.xor(new boolean[] { true, true }));
assertTrue(
"True result for (false, false)",
! BooleanUtils.xor(new boolean[] { false, false }));
assertTrue(
"False result for (true, false)",
BooleanUtils.xor(new boolean[] { true, false }));
assertTrue(
"False result for (false, true)",
BooleanUtils.xor(new boolean[] { false, true }));
}
public void testXor_primitive_validInput_3items() {
assertTrue(
"False result for (false, false, true)",
BooleanUtils.xor(new boolean[] { false, false, true }));
assertTrue(
"False result for (false, true, false)",
BooleanUtils.xor(new boolean[] { false, true, false }));
assertTrue(
"False result for (true, false, false)",
BooleanUtils.xor(new boolean[] { true, false, false }));
assertTrue(
"True result for (true, true, true)",
! BooleanUtils.xor(new boolean[] { true, true, true }));
assertTrue(
"True result for (false, false)",
! BooleanUtils.xor(new boolean[] { false, false, false }));
assertTrue(
"True result for (true, true, false)",
! BooleanUtils.xor(new boolean[] { true, true, false }));
assertTrue(
"True result for (true, false, true)",
! BooleanUtils.xor(new boolean[] { true, false, true }));
assertTrue(
"False result for (false, true, true)",
! BooleanUtils.xor(new boolean[] { false, true, true }));
}
public void testXor_object_nullInput() {
final Boolean[] b = null;
try {
BooleanUtils.xor(b);
fail("Exception was not thrown for null input.");
} catch (IllegalArgumentException ex) {}
}
public void testXor_object_emptyInput() {
try {
BooleanUtils.xor(new Boolean[] {});
fail("Exception was not thrown for empty input.");
} catch (IllegalArgumentException ex) {}
}
public void testXor_object_nullElementInput() {
try {
BooleanUtils.xor(new Boolean[] {null});
fail("Exception was not thrown for null element input.");
} catch (IllegalArgumentException ex) {}
}
public void testXor_object_validInput_2items() {
assertTrue(
"True result for (true, true)",
! BooleanUtils
.xor(new Boolean[] { Boolean.TRUE, Boolean.TRUE })
.booleanValue());
assertTrue(
"True result for (false, false)",
! BooleanUtils
.xor(new Boolean[] { Boolean.FALSE, Boolean.FALSE })
.booleanValue());
assertTrue(
"False result for (true, false)",
BooleanUtils
.xor(new Boolean[] { Boolean.TRUE, Boolean.FALSE })
.booleanValue());
assertTrue(
"False result for (false, true)",
BooleanUtils
.xor(new Boolean[] { Boolean.FALSE, Boolean.TRUE })
.booleanValue());
}
public void testXor_object_validInput_3items() {
assertTrue(
"False result for (false, false, true)",
BooleanUtils
.xor(
new Boolean[] {
Boolean.FALSE,
Boolean.FALSE,
Boolean.TRUE })
.booleanValue());
assertTrue(
"False result for (false, true, false)",
BooleanUtils
.xor(
new Boolean[] {
Boolean.FALSE,
Boolean.TRUE,
Boolean.FALSE })
.booleanValue());
assertTrue(
"False result for (true, false, false)",
BooleanUtils
.xor(
new Boolean[] {
Boolean.TRUE,
Boolean.FALSE,
Boolean.FALSE })
.booleanValue());
assertTrue(
"True result for (true, true, true)",
! BooleanUtils
.xor(new Boolean[] { Boolean.TRUE, Boolean.TRUE, Boolean.TRUE })
.booleanValue());
assertTrue(
"True result for (false, false)",
! BooleanUtils.xor(
new Boolean[] {
Boolean.FALSE,
Boolean.FALSE,
Boolean.FALSE })
.booleanValue());
assertTrue(
"True result for (true, true, false)",
! BooleanUtils.xor(
new Boolean[] {
Boolean.TRUE,
Boolean.TRUE,
Boolean.FALSE })
.booleanValue());
assertTrue(
"True result for (true, false, true)",
! BooleanUtils.xor(
new Boolean[] {
Boolean.TRUE,
Boolean.FALSE,
Boolean.TRUE })
.booleanValue());
assertTrue(
"False result for (false, true, true)",
! BooleanUtils.xor(
new Boolean[] {
Boolean.FALSE,
Boolean.TRUE,
Boolean.TRUE })
.booleanValue());
}
} | // You are a professional Java test case writer, please create a test case named `test_toBoolean_String` for the issue `Lang-LANG-365`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Lang-LANG-365
//
// ## Issue-Title:
// BooleanUtils.toBoolean() - invalid drop-thru in case statement causes StringIndexOutOfBoundsException
//
// ## Issue-Description:
//
// The method BooleanUtils.toBoolean() has a case statement; case 3 drops through to case 4; this can cause StringIndexOutOfBoundsException, for example with the test:
//
//
// assertEquals(false, BooleanUtils.toBoolean("tru"));
//
//
// The end of case 3 should return false.
//
//
// Patch to follow for source and unit test.
//
//
//
//
//
public void test_toBoolean_String() {
| 334 | //----------------------------------------------------------------------- | 51 | 294 | src/test/org/apache/commons/lang/BooleanUtilsTest.java | src/test | ```markdown
## Issue-ID: Lang-LANG-365
## Issue-Title:
BooleanUtils.toBoolean() - invalid drop-thru in case statement causes StringIndexOutOfBoundsException
## Issue-Description:
The method BooleanUtils.toBoolean() has a case statement; case 3 drops through to case 4; this can cause StringIndexOutOfBoundsException, for example with the test:
assertEquals(false, BooleanUtils.toBoolean("tru"));
The end of case 3 should return false.
Patch to follow for source and unit test.
```
You are a professional Java test case writer, please create a test case named `test_toBoolean_String` for the issue `Lang-LANG-365`, utilizing the provided issue report information and the following function signature.
```java
public void test_toBoolean_String() {
```
| 294 | [
"org.apache.commons.lang.BooleanUtils"
] | a598282942b6cce947b47440e6c6fc89daddc22fd6a5b8f0a2a8fd3406f46ad7 | public void test_toBoolean_String() | // You are a professional Java test case writer, please create a test case named `test_toBoolean_String` for the issue `Lang-LANG-365`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Lang-LANG-365
//
// ## Issue-Title:
// BooleanUtils.toBoolean() - invalid drop-thru in case statement causes StringIndexOutOfBoundsException
//
// ## Issue-Description:
//
// The method BooleanUtils.toBoolean() has a case statement; case 3 drops through to case 4; this can cause StringIndexOutOfBoundsException, for example with the test:
//
//
// assertEquals(false, BooleanUtils.toBoolean("tru"));
//
//
// The end of case 3 should return false.
//
//
// Patch to follow for source and unit test.
//
//
//
//
//
| Lang | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.lang;
import java.lang.reflect.Constructor;
import java.lang.reflect.Modifier;
import junit.framework.Test;
import junit.framework.TestCase;
import junit.framework.TestSuite;
import junit.textui.TestRunner;
/**
* Unit tests {@link org.apache.commons.lang.BooleanUtils}.
*
* @author Stephen Colebourne
* @author Matthew Hawthorne
* @version $Id$
*/
public class BooleanUtilsTest extends TestCase {
public BooleanUtilsTest(String name) {
super(name);
}
public static void main(String[] args) {
TestRunner.run(suite());
}
public static Test suite() {
TestSuite suite = new TestSuite(BooleanUtilsTest.class);
suite.setName("BooleanUtils Tests");
return suite;
}
protected void setUp() throws Exception {
super.setUp();
}
protected void tearDown() throws Exception {
super.tearDown();
}
//-----------------------------------------------------------------------
public void testConstructor() {
assertNotNull(new BooleanUtils());
Constructor[] cons = BooleanUtils.class.getDeclaredConstructors();
assertEquals(1, cons.length);
assertEquals(true, Modifier.isPublic(cons[0].getModifiers()));
assertEquals(true, Modifier.isPublic(BooleanUtils.class.getModifiers()));
assertEquals(false, Modifier.isFinal(BooleanUtils.class.getModifiers()));
}
//-----------------------------------------------------------------------
public void test_negate_Boolean() {
assertSame(null, BooleanUtils.negate(null));
assertSame(Boolean.TRUE, BooleanUtils.negate(Boolean.FALSE));
assertSame(Boolean.FALSE, BooleanUtils.negate(Boolean.TRUE));
}
//-----------------------------------------------------------------------
public void test_isTrue_Boolean() {
assertEquals(true, BooleanUtils.isTrue(Boolean.TRUE));
assertEquals(false, BooleanUtils.isTrue(Boolean.FALSE));
assertEquals(false, BooleanUtils.isTrue((Boolean) null));
}
public void test_isNotTrue_Boolean() {
assertEquals(false, BooleanUtils.isNotTrue(Boolean.TRUE));
assertEquals(true, BooleanUtils.isNotTrue(Boolean.FALSE));
assertEquals(true, BooleanUtils.isNotTrue((Boolean) null));
}
//-----------------------------------------------------------------------
public void test_isFalse_Boolean() {
assertEquals(false, BooleanUtils.isFalse(Boolean.TRUE));
assertEquals(true, BooleanUtils.isFalse(Boolean.FALSE));
assertEquals(false, BooleanUtils.isFalse((Boolean) null));
}
public void test_isNotFalse_Boolean() {
assertEquals(true, BooleanUtils.isNotFalse(Boolean.TRUE));
assertEquals(false, BooleanUtils.isNotFalse(Boolean.FALSE));
assertEquals(true, BooleanUtils.isNotFalse((Boolean) null));
}
//-----------------------------------------------------------------------
public void test_toBooleanObject_boolean() {
assertSame(Boolean.TRUE, BooleanUtils.toBooleanObject(true));
assertSame(Boolean.FALSE, BooleanUtils.toBooleanObject(false));
}
public void test_toBoolean_Boolean() {
assertEquals(true, BooleanUtils.toBoolean(Boolean.TRUE));
assertEquals(false, BooleanUtils.toBoolean(Boolean.FALSE));
assertEquals(false, BooleanUtils.toBoolean((Boolean) null));
}
public void test_toBooleanDefaultIfNull_Boolean_boolean() {
assertEquals(true, BooleanUtils.toBooleanDefaultIfNull(Boolean.TRUE, true));
assertEquals(true, BooleanUtils.toBooleanDefaultIfNull(Boolean.TRUE, false));
assertEquals(false, BooleanUtils.toBooleanDefaultIfNull(Boolean.FALSE, true));
assertEquals(false, BooleanUtils.toBooleanDefaultIfNull(Boolean.FALSE, false));
assertEquals(true, BooleanUtils.toBooleanDefaultIfNull((Boolean) null, true));
assertEquals(false, BooleanUtils.toBooleanDefaultIfNull((Boolean) null, false));
}
//-----------------------------------------------------------------------
//-----------------------------------------------------------------------
public void test_toBoolean_int() {
assertEquals(true, BooleanUtils.toBoolean(1));
assertEquals(true, BooleanUtils.toBoolean(-1));
assertEquals(false, BooleanUtils.toBoolean(0));
}
public void test_toBooleanObject_int() {
assertEquals(Boolean.TRUE, BooleanUtils.toBooleanObject(1));
assertEquals(Boolean.TRUE, BooleanUtils.toBooleanObject(-1));
assertEquals(Boolean.FALSE, BooleanUtils.toBooleanObject(0));
}
public void test_toBooleanObject_Integer() {
assertEquals(Boolean.TRUE, BooleanUtils.toBooleanObject(new Integer(1)));
assertEquals(Boolean.TRUE, BooleanUtils.toBooleanObject(new Integer(-1)));
assertEquals(Boolean.FALSE, BooleanUtils.toBooleanObject(new Integer(0)));
assertEquals(null, BooleanUtils.toBooleanObject((Integer) null));
}
//-----------------------------------------------------------------------
public void test_toBoolean_int_int_int() {
assertEquals(true, BooleanUtils.toBoolean(6, 6, 7));
assertEquals(false, BooleanUtils.toBoolean(7, 6, 7));
try {
BooleanUtils.toBoolean(8, 6, 7);
fail();
} catch (IllegalArgumentException ex) {}
}
public void test_toBoolean_Integer_Integer_Integer() {
Integer six = new Integer(6);
Integer seven = new Integer(7);
assertEquals(true, BooleanUtils.toBoolean((Integer) null, null, seven));
assertEquals(false, BooleanUtils.toBoolean((Integer) null, six, null));
try {
BooleanUtils.toBoolean(null, six, seven);
fail();
} catch (IllegalArgumentException ex) {}
assertEquals(true, BooleanUtils.toBoolean(new Integer(6), six, seven));
assertEquals(false, BooleanUtils.toBoolean(new Integer(7), six, seven));
try {
BooleanUtils.toBoolean(new Integer(8), six, seven);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void test_toBooleanObject_int_int_int() {
assertEquals(Boolean.TRUE, BooleanUtils.toBooleanObject(6, 6, 7, 8));
assertEquals(Boolean.FALSE, BooleanUtils.toBooleanObject(7, 6, 7, 8));
assertEquals(null, BooleanUtils.toBooleanObject(8, 6, 7, 8));
try {
BooleanUtils.toBooleanObject(9, 6, 7, 8);
fail();
} catch (IllegalArgumentException ex) {}
}
public void test_toBooleanObject_Integer_Integer_Integer_Integer() {
Integer six = new Integer(6);
Integer seven = new Integer(7);
Integer eight = new Integer(8);
assertSame(Boolean.TRUE, BooleanUtils.toBooleanObject((Integer) null, null, seven, eight));
assertSame(Boolean.FALSE, BooleanUtils.toBooleanObject((Integer) null, six, null, eight));
assertSame(null, BooleanUtils.toBooleanObject((Integer) null, six, seven, null));
try {
BooleanUtils.toBooleanObject(null, six, seven, eight);
fail();
} catch (IllegalArgumentException ex) {}
assertEquals(Boolean.TRUE, BooleanUtils.toBooleanObject(new Integer(6), six, seven, eight));
assertEquals(Boolean.FALSE, BooleanUtils.toBooleanObject(new Integer(7), six, seven, eight));
assertEquals(null, BooleanUtils.toBooleanObject(new Integer(8), six, seven, eight));
try {
BooleanUtils.toBooleanObject(new Integer(9), six, seven, eight);
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void test_toInteger_boolean() {
assertEquals(1, BooleanUtils.toInteger(true));
assertEquals(0, BooleanUtils.toInteger(false));
}
public void test_toIntegerObject_boolean() {
assertEquals(new Integer(1), BooleanUtils.toIntegerObject(true));
assertEquals(new Integer(0), BooleanUtils.toIntegerObject(false));
}
public void test_toIntegerObject_Boolean() {
assertEquals(new Integer(1), BooleanUtils.toIntegerObject(Boolean.TRUE));
assertEquals(new Integer(0), BooleanUtils.toIntegerObject(Boolean.FALSE));
assertEquals(null, BooleanUtils.toIntegerObject((Boolean) null));
}
//-----------------------------------------------------------------------
public void test_toInteger_boolean_int_int() {
assertEquals(6, BooleanUtils.toInteger(true, 6, 7));
assertEquals(7, BooleanUtils.toInteger(false, 6, 7));
}
public void test_toInteger_Boolean_int_int_int() {
assertEquals(6, BooleanUtils.toInteger(Boolean.TRUE, 6, 7, 8));
assertEquals(7, BooleanUtils.toInteger(Boolean.FALSE, 6, 7, 8));
assertEquals(8, BooleanUtils.toInteger(null, 6, 7, 8));
}
public void test_toIntegerObject_boolean_Integer_Integer() {
Integer six = new Integer(6);
Integer seven = new Integer(7);
assertEquals(six, BooleanUtils.toIntegerObject(true, six, seven));
assertEquals(seven, BooleanUtils.toIntegerObject(false, six, seven));
}
public void test_toIntegerObject_Boolean_Integer_Integer_Integer() {
Integer six = new Integer(6);
Integer seven = new Integer(7);
Integer eight = new Integer(8);
assertEquals(six, BooleanUtils.toIntegerObject(Boolean.TRUE, six, seven, eight));
assertEquals(seven, BooleanUtils.toIntegerObject(Boolean.FALSE, six, seven, eight));
assertEquals(eight, BooleanUtils.toIntegerObject((Boolean) null, six, seven, eight));
assertEquals(null, BooleanUtils.toIntegerObject((Boolean) null, six, seven, null));
}
//-----------------------------------------------------------------------
//-----------------------------------------------------------------------
public void test_toBooleanObject_String() {
assertEquals(null, BooleanUtils.toBooleanObject((String) null));
assertEquals(null, BooleanUtils.toBooleanObject(""));
assertEquals(Boolean.FALSE, BooleanUtils.toBooleanObject("false"));
assertEquals(Boolean.FALSE, BooleanUtils.toBooleanObject("no"));
assertEquals(Boolean.FALSE, BooleanUtils.toBooleanObject("off"));
assertEquals(Boolean.FALSE, BooleanUtils.toBooleanObject("FALSE"));
assertEquals(Boolean.FALSE, BooleanUtils.toBooleanObject("NO"));
assertEquals(Boolean.FALSE, BooleanUtils.toBooleanObject("OFF"));
assertEquals(null, BooleanUtils.toBooleanObject("oof"));
assertEquals(Boolean.TRUE, BooleanUtils.toBooleanObject("true"));
assertEquals(Boolean.TRUE, BooleanUtils.toBooleanObject("yes"));
assertEquals(Boolean.TRUE, BooleanUtils.toBooleanObject("on"));
assertEquals(Boolean.TRUE, BooleanUtils.toBooleanObject("TRUE"));
assertEquals(Boolean.TRUE, BooleanUtils.toBooleanObject("ON"));
assertEquals(Boolean.TRUE, BooleanUtils.toBooleanObject("YES"));
assertEquals(Boolean.TRUE, BooleanUtils.toBooleanObject("TruE"));
}
public void test_toBooleanObject_String_String_String_String() {
assertSame(Boolean.TRUE, BooleanUtils.toBooleanObject((String) null, null, "N", "U"));
assertSame(Boolean.FALSE, BooleanUtils.toBooleanObject((String) null, "Y", null, "U"));
assertSame(null, BooleanUtils.toBooleanObject((String) null, "Y", "N", null));
try {
BooleanUtils.toBooleanObject((String) null, "Y", "N", "U");
fail();
} catch (IllegalArgumentException ex) {}
assertEquals(Boolean.TRUE, BooleanUtils.toBooleanObject("Y", "Y", "N", "U"));
assertEquals(Boolean.FALSE, BooleanUtils.toBooleanObject("N", "Y", "N", "U"));
assertEquals(null, BooleanUtils.toBooleanObject("U", "Y", "N", "U"));
try {
BooleanUtils.toBooleanObject(null, "Y", "N", "U");
fail();
} catch (IllegalArgumentException ex) {}
try {
BooleanUtils.toBooleanObject("X", "Y", "N", "U");
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void test_toBoolean_String() {
assertEquals(false, BooleanUtils.toBoolean((String) null));
assertEquals(false, BooleanUtils.toBoolean(""));
assertEquals(false, BooleanUtils.toBoolean("off"));
assertEquals(false, BooleanUtils.toBoolean("oof"));
assertEquals(false, BooleanUtils.toBoolean("yep"));
assertEquals(false, BooleanUtils.toBoolean("trux"));
assertEquals(false, BooleanUtils.toBoolean("false"));
assertEquals(false, BooleanUtils.toBoolean("a"));
assertEquals(true, BooleanUtils.toBoolean("true")); // interned handled differently
assertEquals(true, BooleanUtils.toBoolean(new StringBuffer("tr").append("ue").toString()));
assertEquals(true, BooleanUtils.toBoolean("truE"));
assertEquals(true, BooleanUtils.toBoolean("trUe"));
assertEquals(true, BooleanUtils.toBoolean("trUE"));
assertEquals(true, BooleanUtils.toBoolean("tRue"));
assertEquals(true, BooleanUtils.toBoolean("tRuE"));
assertEquals(true, BooleanUtils.toBoolean("tRUe"));
assertEquals(true, BooleanUtils.toBoolean("tRUE"));
assertEquals(true, BooleanUtils.toBoolean("TRUE"));
assertEquals(true, BooleanUtils.toBoolean("TRUe"));
assertEquals(true, BooleanUtils.toBoolean("TRuE"));
assertEquals(true, BooleanUtils.toBoolean("TRue"));
assertEquals(true, BooleanUtils.toBoolean("TrUE"));
assertEquals(true, BooleanUtils.toBoolean("TrUe"));
assertEquals(true, BooleanUtils.toBoolean("TruE"));
assertEquals(true, BooleanUtils.toBoolean("True"));
assertEquals(true, BooleanUtils.toBoolean("on"));
assertEquals(true, BooleanUtils.toBoolean("oN"));
assertEquals(true, BooleanUtils.toBoolean("On"));
assertEquals(true, BooleanUtils.toBoolean("ON"));
assertEquals(true, BooleanUtils.toBoolean("yes"));
assertEquals(true, BooleanUtils.toBoolean("yeS"));
assertEquals(true, BooleanUtils.toBoolean("yEs"));
assertEquals(true, BooleanUtils.toBoolean("yES"));
assertEquals(true, BooleanUtils.toBoolean("Yes"));
assertEquals(true, BooleanUtils.toBoolean("YeS"));
assertEquals(true, BooleanUtils.toBoolean("YEs"));
assertEquals(true, BooleanUtils.toBoolean("YES"));
assertEquals(false, BooleanUtils.toBoolean("yes?"));
assertEquals(false, BooleanUtils.toBoolean("tru"));
}
public void test_toBoolean_String_String_String() {
assertEquals(true, BooleanUtils.toBoolean((String) null, null, "N"));
assertEquals(false, BooleanUtils.toBoolean((String) null, "Y", null));
try {
BooleanUtils.toBooleanObject((String) null, "Y", "N", "U");
fail();
} catch (IllegalArgumentException ex) {}
assertEquals(true, BooleanUtils.toBoolean("Y", "Y", "N"));
assertEquals(false, BooleanUtils.toBoolean("N", "Y", "N"));
try {
BooleanUtils.toBoolean(null, "Y", "N");
fail();
} catch (IllegalArgumentException ex) {}
try {
BooleanUtils.toBoolean("X", "Y", "N");
fail();
} catch (IllegalArgumentException ex) {}
}
//-----------------------------------------------------------------------
public void test_toStringTrueFalse_Boolean() {
assertEquals(null, BooleanUtils.toStringTrueFalse((Boolean) null));
assertEquals("true", BooleanUtils.toStringTrueFalse(Boolean.TRUE));
assertEquals("false", BooleanUtils.toStringTrueFalse(Boolean.FALSE));
}
public void test_toStringOnOff_Boolean() {
assertEquals(null, BooleanUtils.toStringOnOff((Boolean) null));
assertEquals("on", BooleanUtils.toStringOnOff(Boolean.TRUE));
assertEquals("off", BooleanUtils.toStringOnOff(Boolean.FALSE));
}
public void test_toStringYesNo_Boolean() {
assertEquals(null, BooleanUtils.toStringYesNo((Boolean) null));
assertEquals("yes", BooleanUtils.toStringYesNo(Boolean.TRUE));
assertEquals("no", BooleanUtils.toStringYesNo(Boolean.FALSE));
}
public void test_toString_Boolean_String_String_String() {
assertEquals("U", BooleanUtils.toString((Boolean) null, "Y", "N", "U"));
assertEquals("Y", BooleanUtils.toString(Boolean.TRUE, "Y", "N", "U"));
assertEquals("N", BooleanUtils.toString(Boolean.FALSE, "Y", "N", "U"));
}
//-----------------------------------------------------------------------
public void test_toStringTrueFalse_boolean() {
assertEquals("true", BooleanUtils.toStringTrueFalse(true));
assertEquals("false", BooleanUtils.toStringTrueFalse(false));
}
public void test_toStringOnOff_boolean() {
assertEquals("on", BooleanUtils.toStringOnOff(true));
assertEquals("off", BooleanUtils.toStringOnOff(false));
}
public void test_toStringYesNo_boolean() {
assertEquals("yes", BooleanUtils.toStringYesNo(true));
assertEquals("no", BooleanUtils.toStringYesNo(false));
}
public void test_toString_boolean_String_String_String() {
assertEquals("Y", BooleanUtils.toString(true, "Y", "N"));
assertEquals("N", BooleanUtils.toString(false, "Y", "N"));
}
// testXor
// -----------------------------------------------------------------------
public void testXor_primitive_nullInput() {
final boolean[] b = null;
try {
BooleanUtils.xor(b);
fail("Exception was not thrown for null input.");
} catch (IllegalArgumentException ex) {}
}
public void testXor_primitive_emptyInput() {
try {
BooleanUtils.xor(new boolean[] {});
fail("Exception was not thrown for empty input.");
} catch (IllegalArgumentException ex) {}
}
public void testXor_primitive_validInput_2items() {
assertTrue(
"True result for (true, true)",
! BooleanUtils.xor(new boolean[] { true, true }));
assertTrue(
"True result for (false, false)",
! BooleanUtils.xor(new boolean[] { false, false }));
assertTrue(
"False result for (true, false)",
BooleanUtils.xor(new boolean[] { true, false }));
assertTrue(
"False result for (false, true)",
BooleanUtils.xor(new boolean[] { false, true }));
}
public void testXor_primitive_validInput_3items() {
assertTrue(
"False result for (false, false, true)",
BooleanUtils.xor(new boolean[] { false, false, true }));
assertTrue(
"False result for (false, true, false)",
BooleanUtils.xor(new boolean[] { false, true, false }));
assertTrue(
"False result for (true, false, false)",
BooleanUtils.xor(new boolean[] { true, false, false }));
assertTrue(
"True result for (true, true, true)",
! BooleanUtils.xor(new boolean[] { true, true, true }));
assertTrue(
"True result for (false, false)",
! BooleanUtils.xor(new boolean[] { false, false, false }));
assertTrue(
"True result for (true, true, false)",
! BooleanUtils.xor(new boolean[] { true, true, false }));
assertTrue(
"True result for (true, false, true)",
! BooleanUtils.xor(new boolean[] { true, false, true }));
assertTrue(
"False result for (false, true, true)",
! BooleanUtils.xor(new boolean[] { false, true, true }));
}
public void testXor_object_nullInput() {
final Boolean[] b = null;
try {
BooleanUtils.xor(b);
fail("Exception was not thrown for null input.");
} catch (IllegalArgumentException ex) {}
}
public void testXor_object_emptyInput() {
try {
BooleanUtils.xor(new Boolean[] {});
fail("Exception was not thrown for empty input.");
} catch (IllegalArgumentException ex) {}
}
public void testXor_object_nullElementInput() {
try {
BooleanUtils.xor(new Boolean[] {null});
fail("Exception was not thrown for null element input.");
} catch (IllegalArgumentException ex) {}
}
public void testXor_object_validInput_2items() {
assertTrue(
"True result for (true, true)",
! BooleanUtils
.xor(new Boolean[] { Boolean.TRUE, Boolean.TRUE })
.booleanValue());
assertTrue(
"True result for (false, false)",
! BooleanUtils
.xor(new Boolean[] { Boolean.FALSE, Boolean.FALSE })
.booleanValue());
assertTrue(
"False result for (true, false)",
BooleanUtils
.xor(new Boolean[] { Boolean.TRUE, Boolean.FALSE })
.booleanValue());
assertTrue(
"False result for (false, true)",
BooleanUtils
.xor(new Boolean[] { Boolean.FALSE, Boolean.TRUE })
.booleanValue());
}
public void testXor_object_validInput_3items() {
assertTrue(
"False result for (false, false, true)",
BooleanUtils
.xor(
new Boolean[] {
Boolean.FALSE,
Boolean.FALSE,
Boolean.TRUE })
.booleanValue());
assertTrue(
"False result for (false, true, false)",
BooleanUtils
.xor(
new Boolean[] {
Boolean.FALSE,
Boolean.TRUE,
Boolean.FALSE })
.booleanValue());
assertTrue(
"False result for (true, false, false)",
BooleanUtils
.xor(
new Boolean[] {
Boolean.TRUE,
Boolean.FALSE,
Boolean.FALSE })
.booleanValue());
assertTrue(
"True result for (true, true, true)",
! BooleanUtils
.xor(new Boolean[] { Boolean.TRUE, Boolean.TRUE, Boolean.TRUE })
.booleanValue());
assertTrue(
"True result for (false, false)",
! BooleanUtils.xor(
new Boolean[] {
Boolean.FALSE,
Boolean.FALSE,
Boolean.FALSE })
.booleanValue());
assertTrue(
"True result for (true, true, false)",
! BooleanUtils.xor(
new Boolean[] {
Boolean.TRUE,
Boolean.TRUE,
Boolean.FALSE })
.booleanValue());
assertTrue(
"True result for (true, false, true)",
! BooleanUtils.xor(
new Boolean[] {
Boolean.TRUE,
Boolean.FALSE,
Boolean.TRUE })
.booleanValue());
assertTrue(
"False result for (false, true, true)",
! BooleanUtils.xor(
new Boolean[] {
Boolean.FALSE,
Boolean.TRUE,
Boolean.TRUE })
.booleanValue());
}
} |
|
public void testIndexOfLang294() {
StrBuilder sb = new StrBuilder("onetwothree");
sb.deleteFirst("three");
assertEquals(-1, sb.indexOf("three"));
} | org.apache.commons.lang.text.StrBuilderTest::testIndexOfLang294 | src/test/org/apache/commons/lang/text/StrBuilderTest.java | 1,741 | src/test/org/apache/commons/lang/text/StrBuilderTest.java | testIndexOfLang294 | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.lang.text;
import java.io.Reader;
import java.io.Writer;
import java.util.Arrays;
import junit.framework.Test;
import junit.framework.TestCase;
import junit.framework.TestSuite;
import junit.textui.TestRunner;
import org.apache.commons.lang.ArrayUtils;
/**
* Unit tests for {@link org.apache.commons.lang.text.StrBuilder}.
*
* @author Michael Heuer
* @version $Id$
*/
public class StrBuilderTest extends TestCase {
/**
* Main method.
*
* @param args command line arguments, ignored
*/
public static void main(String[] args) {
TestRunner.run(suite());
}
/**
* Return a new test suite containing this test case.
*
* @return a new test suite containing this test case
*/
public static Test suite() {
TestSuite suite = new TestSuite(StrBuilderTest.class);
suite.setName("StrBuilder Tests");
return suite;
}
/**
* Create a new test case with the specified name.
*
* @param name
* name
*/
public StrBuilderTest(String name) {
super(name);
}
//-----------------------------------------------------------------------
public void testConstructors() {
StrBuilder sb0 = new StrBuilder();
assertEquals(32, sb0.capacity());
assertEquals(0, sb0.length());
assertEquals(0, sb0.size());
StrBuilder sb1 = new StrBuilder(32);
assertEquals(32, sb1.capacity());
assertEquals(0, sb1.length());
assertEquals(0, sb1.size());
StrBuilder sb2 = new StrBuilder(0);
assertEquals(32, sb2.capacity());
assertEquals(0, sb2.length());
assertEquals(0, sb2.size());
StrBuilder sb3 = new StrBuilder(-1);
assertEquals(32, sb3.capacity());
assertEquals(0, sb3.length());
assertEquals(0, sb3.size());
StrBuilder sb4 = new StrBuilder(1);
assertEquals(1, sb4.capacity());
assertEquals(0, sb4.length());
assertEquals(0, sb4.size());
StrBuilder sb5 = new StrBuilder((String) null);
assertEquals(32, sb5.capacity());
assertEquals(0, sb5.length());
assertEquals(0, sb5.size());
StrBuilder sb6 = new StrBuilder("");
assertEquals(32, sb6.capacity());
assertEquals(0, sb6.length());
assertEquals(0, sb6.size());
StrBuilder sb7 = new StrBuilder("foo");
assertEquals(35, sb7.capacity());
assertEquals(3, sb7.length());
assertEquals(3, sb7.size());
}
//-----------------------------------------------------------------------
public void testChaining() {
StrBuilder sb = new StrBuilder();
assertSame(sb, sb.setNewLineText(null));
assertSame(sb, sb.setNullText(null));
assertSame(sb, sb.setLength(1));
assertSame(sb, sb.setCharAt(0, 'a'));
assertSame(sb, sb.ensureCapacity(0));
assertSame(sb, sb.minimizeCapacity());
assertSame(sb, sb.clear());
assertSame(sb, sb.reverse());
assertSame(sb, sb.trim());
}
//-----------------------------------------------------------------------
public void testGetSetNewLineText() {
StrBuilder sb = new StrBuilder();
assertEquals(null, sb.getNewLineText());
sb.setNewLineText("#");
assertEquals("#", sb.getNewLineText());
sb.setNewLineText("");
assertEquals("", sb.getNewLineText());
sb.setNewLineText((String) null);
assertEquals(null, sb.getNewLineText());
}
//-----------------------------------------------------------------------
public void testGetSetNullText() {
StrBuilder sb = new StrBuilder();
assertEquals(null, sb.getNullText());
sb.setNullText("null");
assertEquals("null", sb.getNullText());
sb.setNullText("");
assertEquals(null, sb.getNullText());
sb.setNullText("NULL");
assertEquals("NULL", sb.getNullText());
sb.setNullText((String) null);
assertEquals(null, sb.getNullText());
}
//-----------------------------------------------------------------------
public void testCapacityAndLength() {
StrBuilder sb = new StrBuilder();
assertEquals(32, sb.capacity());
assertEquals(0, sb.length());
assertEquals(0, sb.size());
assertTrue(sb.isEmpty());
sb.minimizeCapacity();
assertEquals(0, sb.capacity());
assertEquals(0, sb.length());
assertEquals(0, sb.size());
assertTrue(sb.isEmpty());
sb.ensureCapacity(32);
assertTrue(sb.capacity() >= 32);
assertEquals(0, sb.length());
assertEquals(0, sb.size());
assertTrue(sb.isEmpty());
sb.append("foo");
assertTrue(sb.capacity() >= 32);
assertEquals(3, sb.length());
assertEquals(3, sb.size());
assertTrue(sb.isEmpty() == false);
sb.clear();
assertTrue(sb.capacity() >= 32);
assertEquals(0, sb.length());
assertEquals(0, sb.size());
assertTrue(sb.isEmpty());
sb.append("123456789012345678901234567890123");
assertTrue(sb.capacity() > 32);
assertEquals(33, sb.length());
assertEquals(33, sb.size());
assertTrue(sb.isEmpty() == false);
sb.ensureCapacity(16);
assertTrue(sb.capacity() > 16);
assertEquals(33, sb.length());
assertEquals(33, sb.size());
assertTrue(sb.isEmpty() == false);
sb.minimizeCapacity();
assertEquals(33, sb.capacity());
assertEquals(33, sb.length());
assertEquals(33, sb.size());
assertTrue(sb.isEmpty() == false);
try {
sb.setLength(-1);
fail("setLength(-1) expected StringIndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {
// expected
}
sb.setLength(33);
assertEquals(33, sb.capacity());
assertEquals(33, sb.length());
assertEquals(33, sb.size());
assertTrue(sb.isEmpty() == false);
sb.setLength(16);
assertTrue(sb.capacity() >= 16);
assertEquals(16, sb.length());
assertEquals(16, sb.size());
assertEquals("1234567890123456", sb.toString());
assertTrue(sb.isEmpty() == false);
sb.setLength(32);
assertTrue(sb.capacity() >= 32);
assertEquals(32, sb.length());
assertEquals(32, sb.size());
assertEquals("1234567890123456\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0", sb.toString());
assertTrue(sb.isEmpty() == false);
sb.setLength(0);
assertTrue(sb.capacity() >= 32);
assertEquals(0, sb.length());
assertEquals(0, sb.size());
assertTrue(sb.isEmpty());
}
//-----------------------------------------------------------------------
public void testLength() {
StrBuilder sb = new StrBuilder();
assertEquals(0, sb.length());
sb.append("Hello");
assertEquals(5, sb.length());
}
public void testSetLength() {
StrBuilder sb = new StrBuilder();
sb.append("Hello");
sb.setLength(2); // shorten
assertEquals("He", sb.toString());
sb.setLength(2); // no change
assertEquals("He", sb.toString());
sb.setLength(3); // lengthen
assertEquals("He\0", sb.toString());
try {
sb.setLength(-1);
fail("setLength(-1) expected StringIndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {
// expected
}
}
//-----------------------------------------------------------------------
public void testCapacity() {
StrBuilder sb = new StrBuilder();
assertEquals(sb.buffer.length, sb.capacity());
sb.append("HelloWorldHelloWorldHelloWorldHelloWorld");
assertEquals(sb.buffer.length, sb.capacity());
}
public void testEnsureCapacity() {
StrBuilder sb = new StrBuilder();
sb.ensureCapacity(2);
assertEquals(true, sb.capacity() >= 2);
sb.ensureCapacity(-1);
assertEquals(true, sb.capacity() >= 0);
sb.append("HelloWorld");
sb.ensureCapacity(40);
assertEquals(true, sb.capacity() >= 40);
}
public void testMinimizeCapacity() {
StrBuilder sb = new StrBuilder();
sb.minimizeCapacity();
assertEquals(0, sb.capacity());
sb.append("HelloWorld");
sb.minimizeCapacity();
assertEquals(10, sb.capacity());
}
//-----------------------------------------------------------------------
public void testSize() {
StrBuilder sb = new StrBuilder();
assertEquals(0, sb.size());
sb.append("Hello");
assertEquals(5, sb.size());
}
public void testIsEmpty() {
StrBuilder sb = new StrBuilder();
assertEquals(true, sb.isEmpty());
sb.append("Hello");
assertEquals(false, sb.isEmpty());
sb.clear();
assertEquals(true, sb.isEmpty());
}
public void testClear() {
StrBuilder sb = new StrBuilder();
sb.append("Hello");
sb.clear();
assertEquals(0, sb.length());
assertEquals(true, sb.buffer.length >= 5);
}
//-----------------------------------------------------------------------
public void testCharAt() {
StrBuilder sb = new StrBuilder();
try {
sb.charAt(0);
fail("charAt(0) expected IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {
// expected
}
try {
sb.charAt(-1);
fail("charAt(-1) expected IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {
// expected
}
sb.append("foo");
assertEquals('f', sb.charAt(0));
assertEquals('o', sb.charAt(1));
assertEquals('o', sb.charAt(2));
try {
sb.charAt(-1);
fail("charAt(-1) expected IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {
// expected
}
try {
sb.charAt(3);
fail("charAt(3) expected IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {
// expected
}
}
//-----------------------------------------------------------------------
public void testSetCharAt() {
StrBuilder sb = new StrBuilder();
try {
sb.setCharAt(0, 'f');
fail("setCharAt(0,) expected IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {
// expected
}
try {
sb.setCharAt(-1, 'f');
fail("setCharAt(-1,) expected IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {
// expected
}
sb.append("foo");
sb.setCharAt(0, 'b');
sb.setCharAt(1, 'a');
sb.setCharAt(2, 'r');
try {
sb.setCharAt(3, '!');
fail("setCharAt(3,) expected IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {
// expected
}
assertEquals("bar", sb.toString());
}
//-----------------------------------------------------------------------
public void testDeleteCharAt() {
StrBuilder sb = new StrBuilder("abc");
sb.deleteCharAt(0);
assertEquals("bc", sb.toString());
try {
sb.deleteCharAt(1000);
fail("Expected IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {}
}
//-----------------------------------------------------------------------
public void testToCharArray() {
StrBuilder sb = new StrBuilder();
assertEquals(ArrayUtils.EMPTY_CHAR_ARRAY, sb.toCharArray());
char[] a = sb.toCharArray();
assertNotNull("toCharArray() result is null", a);
assertEquals("toCharArray() result is too large", 0, a.length);
sb.append("junit");
a = sb.toCharArray();
assertEquals("toCharArray() result incorrect length", 5, a.length);
assertTrue("toCharArray() result does not match", Arrays.equals("junit".toCharArray(), a));
}
public void testToCharArrayIntInt() {
StrBuilder sb = new StrBuilder();
assertEquals(ArrayUtils.EMPTY_CHAR_ARRAY, sb.toCharArray(0, 0));
sb.append("junit");
char[] a = sb.toCharArray(0, 20); // too large test
assertEquals("toCharArray(int,int) result incorrect length", 5, a.length);
assertTrue("toCharArray(int,int) result does not match", Arrays.equals("junit".toCharArray(), a));
a = sb.toCharArray(0, 4);
assertEquals("toCharArray(int,int) result incorrect length", 4, a.length);
assertTrue("toCharArray(int,int) result does not match", Arrays.equals("juni".toCharArray(), a));
a = sb.toCharArray(0, 4);
assertEquals("toCharArray(int,int) result incorrect length", 4, a.length);
assertTrue("toCharArray(int,int) result does not match", Arrays.equals("juni".toCharArray(), a));
a = sb.toCharArray(0, 1);
assertNotNull("toCharArray(int,int) result is null", a);
try {
sb.toCharArray(-1, 5);
fail("no string index out of bound on -1");
} catch (IndexOutOfBoundsException e) {
}
try {
sb.toCharArray(6, 5);
fail("no string index out of bound on -1");
} catch (IndexOutOfBoundsException e) {
}
}
public void testGetChars ( ) {
StrBuilder sb = new StrBuilder();
char[] input = new char[10];
char[] a = sb.getChars(input);
assertSame (input, a);
assertTrue(Arrays.equals(new char[10], a));
sb.append("junit");
a = sb.getChars(input);
assertSame(input, a);
assertTrue(Arrays.equals(new char[] {'j','u','n','i','t',0,0,0,0,0},a));
a = sb.getChars(null);
assertNotSame(input,a);
assertEquals(5,a.length);
assertTrue(Arrays.equals("junit".toCharArray(),a));
input = new char[5];
a = sb.getChars(input);
assertSame(input, a);
input = new char[4];
a = sb.getChars(input);
assertNotSame(input, a);
}
public void testGetCharsIntIntCharArrayInt( ) {
StrBuilder sb = new StrBuilder();
sb.append("junit");
char[] a = new char[5];
sb.getChars(0,5,a,0);
assertTrue(Arrays.equals(new char[] {'j','u','n','i','t'},a));
a = new char[5];
sb.getChars(0,2,a,3);
assertTrue(Arrays.equals(new char[] {0,0,0,'j','u'},a));
try {
sb.getChars(-1,0,a,0);
fail("no exception");
}
catch (IndexOutOfBoundsException e) {
}
try {
sb.getChars(0,-1,a,0);
fail("no exception");
}
catch (IndexOutOfBoundsException e) {
}
try {
sb.getChars(0,20,a,0);
fail("no exception");
}
catch (IndexOutOfBoundsException e) {
}
try {
sb.getChars(4,2,a,0);
fail("no exception");
}
catch (IndexOutOfBoundsException e) {
}
}
//-----------------------------------------------------------------------
public void testDeleteIntInt() {
StrBuilder sb = new StrBuilder("abc");
sb.delete(0, 1);
assertEquals("bc", sb.toString());
sb.delete(1, 2);
assertEquals("b", sb.toString());
sb.delete(0, 1);
assertEquals("", sb.toString());
sb.delete(0, 1000);
assertEquals("", sb.toString());
try {
sb.delete(1, 2);
fail("Expected IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {}
try {
sb.delete(-1, 1);
fail("Expected IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {}
sb = new StrBuilder("anything");
try {
sb.delete(2, 1);
fail("Expected IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {}
}
//-----------------------------------------------------------------------
public void testDeleteAll_char() {
StrBuilder sb = new StrBuilder("abcbccba");
sb.deleteAll('X');
assertEquals("abcbccba", sb.toString());
sb.deleteAll('a');
assertEquals("bcbccb", sb.toString());
sb.deleteAll('c');
assertEquals("bbb", sb.toString());
sb.deleteAll('b');
assertEquals("", sb.toString());
sb = new StrBuilder("");
sb.deleteAll('b');
assertEquals("", sb.toString());
}
public void testDeleteFirst_char() {
StrBuilder sb = new StrBuilder("abcba");
sb.deleteFirst('X');
assertEquals("abcba", sb.toString());
sb.deleteFirst('a');
assertEquals("bcba", sb.toString());
sb.deleteFirst('c');
assertEquals("bba", sb.toString());
sb.deleteFirst('b');
assertEquals("ba", sb.toString());
sb = new StrBuilder("");
sb.deleteFirst('b');
assertEquals("", sb.toString());
}
// -----------------------------------------------------------------------
public void testDeleteAll_String() {
StrBuilder sb = new StrBuilder("abcbccba");
sb.deleteAll((String) null);
assertEquals("abcbccba", sb.toString());
sb.deleteAll("");
assertEquals("abcbccba", sb.toString());
sb.deleteAll("X");
assertEquals("abcbccba", sb.toString());
sb.deleteAll("a");
assertEquals("bcbccb", sb.toString());
sb.deleteAll("c");
assertEquals("bbb", sb.toString());
sb.deleteAll("b");
assertEquals("", sb.toString());
sb = new StrBuilder("abcbccba");
sb.deleteAll("bc");
assertEquals("acba", sb.toString());
sb = new StrBuilder("");
sb.deleteAll("bc");
assertEquals("", sb.toString());
}
public void testDeleteFirst_String() {
StrBuilder sb = new StrBuilder("abcbccba");
sb.deleteFirst((String) null);
assertEquals("abcbccba", sb.toString());
sb.deleteFirst("");
assertEquals("abcbccba", sb.toString());
sb.deleteFirst("X");
assertEquals("abcbccba", sb.toString());
sb.deleteFirst("a");
assertEquals("bcbccba", sb.toString());
sb.deleteFirst("c");
assertEquals("bbccba", sb.toString());
sb.deleteFirst("b");
assertEquals("bccba", sb.toString());
sb = new StrBuilder("abcbccba");
sb.deleteFirst("bc");
assertEquals("abccba", sb.toString());
sb = new StrBuilder("");
sb.deleteFirst("bc");
assertEquals("", sb.toString());
}
// -----------------------------------------------------------------------
public void testDeleteAll_StrMatcher() {
StrBuilder sb = new StrBuilder("A0xA1A2yA3");
sb.deleteAll((StrMatcher) null);
assertEquals("A0xA1A2yA3", sb.toString());
sb.deleteAll(A_NUMBER_MATCHER);
assertEquals("xy", sb.toString());
sb = new StrBuilder("Ax1");
sb.deleteAll(A_NUMBER_MATCHER);
assertEquals("Ax1", sb.toString());
sb = new StrBuilder("");
sb.deleteAll(A_NUMBER_MATCHER);
assertEquals("", sb.toString());
}
public void testDeleteFirst_StrMatcher() {
StrBuilder sb = new StrBuilder("A0xA1A2yA3");
sb.deleteFirst((StrMatcher) null);
assertEquals("A0xA1A2yA3", sb.toString());
sb.deleteFirst(A_NUMBER_MATCHER);
assertEquals("xA1A2yA3", sb.toString());
sb = new StrBuilder("Ax1");
sb.deleteFirst(A_NUMBER_MATCHER);
assertEquals("Ax1", sb.toString());
sb = new StrBuilder("");
sb.deleteFirst(A_NUMBER_MATCHER);
assertEquals("", sb.toString());
}
// -----------------------------------------------------------------------
public void testReplace_int_int_String() {
StrBuilder sb = new StrBuilder("abc");
sb.replace(0, 1, "d");
assertEquals("dbc", sb.toString());
sb.replace(0, 1, "aaa");
assertEquals("aaabc", sb.toString());
sb.replace(0, 3, "");
assertEquals("bc", sb.toString());
sb.replace(1, 2, (String) null);
assertEquals("b", sb.toString());
sb.replace(1, 1000, "text");
assertEquals("btext", sb.toString());
sb.replace(0, 1000, "text");
assertEquals("text", sb.toString());
sb = new StrBuilder("atext");
sb.replace(1, 1, "ny");
assertEquals("anytext", sb.toString());
try {
sb.replace(2, 1, "anything");
fail("Expected IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {}
sb = new StrBuilder();
try {
sb.replace(1, 2, "anything");
fail("Expected IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {}
try {
sb.replace(-1, 1, "anything");
fail("Expected IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {}
}
//-----------------------------------------------------------------------
public void testReplaceAll_char_char() {
StrBuilder sb = new StrBuilder("abcbccba");
sb.replaceAll('x', 'y');
assertEquals("abcbccba", sb.toString());
sb.replaceAll('a', 'd');
assertEquals("dbcbccbd", sb.toString());
sb.replaceAll('b', 'e');
assertEquals("dececced", sb.toString());
sb.replaceAll('c', 'f');
assertEquals("defeffed", sb.toString());
sb.replaceAll('d', 'd');
assertEquals("defeffed", sb.toString());
}
//-----------------------------------------------------------------------
public void testReplaceFirst_char_char() {
StrBuilder sb = new StrBuilder("abcbccba");
sb.replaceFirst('x', 'y');
assertEquals("abcbccba", sb.toString());
sb.replaceFirst('a', 'd');
assertEquals("dbcbccba", sb.toString());
sb.replaceFirst('b', 'e');
assertEquals("decbccba", sb.toString());
sb.replaceFirst('c', 'f');
assertEquals("defbccba", sb.toString());
sb.replaceFirst('d', 'd');
assertEquals("defbccba", sb.toString());
}
//-----------------------------------------------------------------------
public void testReplaceAll_String_String() {
StrBuilder sb = new StrBuilder("abcbccba");
sb.replaceAll((String) null, null);
assertEquals("abcbccba", sb.toString());
sb.replaceAll((String) null, "anything");
assertEquals("abcbccba", sb.toString());
sb.replaceAll("", null);
assertEquals("abcbccba", sb.toString());
sb.replaceAll("", "anything");
assertEquals("abcbccba", sb.toString());
sb.replaceAll("x", "y");
assertEquals("abcbccba", sb.toString());
sb.replaceAll("a", "d");
assertEquals("dbcbccbd", sb.toString());
sb.replaceAll("d", null);
assertEquals("bcbccb", sb.toString());
sb.replaceAll("cb", "-");
assertEquals("b-c-", sb.toString());
sb = new StrBuilder("abcba");
sb.replaceAll("b", "xbx");
assertEquals("axbxcxbxa", sb.toString());
sb = new StrBuilder("bb");
sb.replaceAll("b", "xbx");
assertEquals("xbxxbx", sb.toString());
}
public void testReplaceFirst_String_String() {
StrBuilder sb = new StrBuilder("abcbccba");
sb.replaceFirst((String) null, null);
assertEquals("abcbccba", sb.toString());
sb.replaceFirst((String) null, "anything");
assertEquals("abcbccba", sb.toString());
sb.replaceFirst("", null);
assertEquals("abcbccba", sb.toString());
sb.replaceFirst("", "anything");
assertEquals("abcbccba", sb.toString());
sb.replaceFirst("x", "y");
assertEquals("abcbccba", sb.toString());
sb.replaceFirst("a", "d");
assertEquals("dbcbccba", sb.toString());
sb.replaceFirst("d", null);
assertEquals("bcbccba", sb.toString());
sb.replaceFirst("cb", "-");
assertEquals("b-ccba", sb.toString());
sb = new StrBuilder("abcba");
sb.replaceFirst("b", "xbx");
assertEquals("axbxcba", sb.toString());
sb = new StrBuilder("bb");
sb.replaceFirst("b", "xbx");
assertEquals("xbxb", sb.toString());
}
//-----------------------------------------------------------------------
public void testReplaceAll_StrMatcher_String() {
StrBuilder sb = new StrBuilder("abcbccba");
sb.replaceAll((StrMatcher) null, null);
assertEquals("abcbccba", sb.toString());
sb.replaceAll((StrMatcher) null, "anything");
assertEquals("abcbccba", sb.toString());
sb.replaceAll(StrMatcher.noneMatcher(), null);
assertEquals("abcbccba", sb.toString());
sb.replaceAll(StrMatcher.noneMatcher(), "anything");
assertEquals("abcbccba", sb.toString());
sb.replaceAll(StrMatcher.charMatcher('x'), "y");
assertEquals("abcbccba", sb.toString());
sb.replaceAll(StrMatcher.charMatcher('a'), "d");
assertEquals("dbcbccbd", sb.toString());
sb.replaceAll(StrMatcher.charMatcher('d'), null);
assertEquals("bcbccb", sb.toString());
sb.replaceAll(StrMatcher.stringMatcher("cb"), "-");
assertEquals("b-c-", sb.toString());
sb = new StrBuilder("abcba");
sb.replaceAll(StrMatcher.charMatcher('b'), "xbx");
assertEquals("axbxcxbxa", sb.toString());
sb = new StrBuilder("bb");
sb.replaceAll(StrMatcher.charMatcher('b'), "xbx");
assertEquals("xbxxbx", sb.toString());
sb = new StrBuilder("A1-A2A3-A4");
sb.replaceAll(A_NUMBER_MATCHER, "***");
assertEquals("***-******-***", sb.toString());
}
public void testReplaceFirst_StrMatcher_String() {
StrBuilder sb = new StrBuilder("abcbccba");
sb.replaceFirst((StrMatcher) null, null);
assertEquals("abcbccba", sb.toString());
sb.replaceFirst((StrMatcher) null, "anything");
assertEquals("abcbccba", sb.toString());
sb.replaceFirst(StrMatcher.noneMatcher(), null);
assertEquals("abcbccba", sb.toString());
sb.replaceFirst(StrMatcher.noneMatcher(), "anything");
assertEquals("abcbccba", sb.toString());
sb.replaceFirst(StrMatcher.charMatcher('x'), "y");
assertEquals("abcbccba", sb.toString());
sb.replaceFirst(StrMatcher.charMatcher('a'), "d");
assertEquals("dbcbccba", sb.toString());
sb.replaceFirst(StrMatcher.charMatcher('d'), null);
assertEquals("bcbccba", sb.toString());
sb.replaceFirst(StrMatcher.stringMatcher("cb"), "-");
assertEquals("b-ccba", sb.toString());
sb = new StrBuilder("abcba");
sb.replaceFirst(StrMatcher.charMatcher('b'), "xbx");
assertEquals("axbxcba", sb.toString());
sb = new StrBuilder("bb");
sb.replaceFirst(StrMatcher.charMatcher('b'), "xbx");
assertEquals("xbxb", sb.toString());
sb = new StrBuilder("A1-A2A3-A4");
sb.replaceFirst(A_NUMBER_MATCHER, "***");
assertEquals("***-A2A3-A4", sb.toString());
}
//-----------------------------------------------------------------------
public void testReplace_StrMatcher_String_int_int_int_VaryMatcher() {
StrBuilder sb = new StrBuilder("abcbccba");
sb.replace((StrMatcher) null, "x", 0, sb.length(), -1);
assertEquals("abcbccba", sb.toString());
sb.replace(StrMatcher.charMatcher('a'), "x", 0, sb.length(), -1);
assertEquals("xbcbccbx", sb.toString());
sb.replace(StrMatcher.stringMatcher("cb"), "x", 0, sb.length(), -1);
assertEquals("xbxcxx", sb.toString());
sb = new StrBuilder("A1-A2A3-A4");
sb.replace(A_NUMBER_MATCHER, "***", 0, sb.length(), -1);
assertEquals("***-******-***", sb.toString());
sb = new StrBuilder();
sb.replace(A_NUMBER_MATCHER, "***", 0, sb.length(), -1);
assertEquals("", sb.toString());
}
public void testReplace_StrMatcher_String_int_int_int_VaryReplace() {
StrBuilder sb = new StrBuilder("abcbccba");
sb.replace(StrMatcher.stringMatcher("cb"), "cb", 0, sb.length(), -1);
assertEquals("abcbccba", sb.toString());
sb = new StrBuilder("abcbccba");
sb.replace(StrMatcher.stringMatcher("cb"), "-", 0, sb.length(), -1);
assertEquals("ab-c-a", sb.toString());
sb = new StrBuilder("abcbccba");
sb.replace(StrMatcher.stringMatcher("cb"), "+++", 0, sb.length(), -1);
assertEquals("ab+++c+++a", sb.toString());
sb = new StrBuilder("abcbccba");
sb.replace(StrMatcher.stringMatcher("cb"), "", 0, sb.length(), -1);
assertEquals("abca", sb.toString());
sb = new StrBuilder("abcbccba");
sb.replace(StrMatcher.stringMatcher("cb"), null, 0, sb.length(), -1);
assertEquals("abca", sb.toString());
}
public void testReplace_StrMatcher_String_int_int_int_VaryStartIndex() {
StrBuilder sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, sb.length(), -1);
assertEquals("-x--y-", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 1, sb.length(), -1);
assertEquals("aax--y-", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 2, sb.length(), -1);
assertEquals("aax--y-", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 3, sb.length(), -1);
assertEquals("aax--y-", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 4, sb.length(), -1);
assertEquals("aaxa-ay-", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 5, sb.length(), -1);
assertEquals("aaxaa-y-", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 6, sb.length(), -1);
assertEquals("aaxaaaay-", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 7, sb.length(), -1);
assertEquals("aaxaaaay-", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 8, sb.length(), -1);
assertEquals("aaxaaaay-", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 9, sb.length(), -1);
assertEquals("aaxaaaayaa", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 10, sb.length(), -1);
assertEquals("aaxaaaayaa", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
try {
sb.replace(StrMatcher.stringMatcher("aa"), "-", 11, sb.length(), -1);
fail();
} catch (IndexOutOfBoundsException ex) {}
assertEquals("aaxaaaayaa", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
try {
sb.replace(StrMatcher.stringMatcher("aa"), "-", -1, sb.length(), -1);
fail();
} catch (IndexOutOfBoundsException ex) {}
assertEquals("aaxaaaayaa", sb.toString());
}
public void testReplace_StrMatcher_String_int_int_int_VaryEndIndex() {
StrBuilder sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 0, -1);
assertEquals("aaxaaaayaa", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 2, -1);
assertEquals("-xaaaayaa", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 3, -1);
assertEquals("-xaaaayaa", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 4, -1);
assertEquals("-xaaaayaa", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 5, -1);
assertEquals("-x-aayaa", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 6, -1);
assertEquals("-x-aayaa", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 7, -1);
assertEquals("-x--yaa", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 8, -1);
assertEquals("-x--yaa", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 9, -1);
assertEquals("-x--yaa", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 10, -1);
assertEquals("-x--y-", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 1000, -1);
assertEquals("-x--y-", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
try {
sb.replace(StrMatcher.stringMatcher("aa"), "-", 2, 1, -1);
fail();
} catch (IndexOutOfBoundsException ex) {}
assertEquals("aaxaaaayaa", sb.toString());
}
public void testReplace_StrMatcher_String_int_int_int_VaryCount() {
StrBuilder sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 10, -1);
assertEquals("-x--y-", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 10, 0);
assertEquals("aaxaaaayaa", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 10, 1);
assertEquals("-xaaaayaa", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 10, 2);
assertEquals("-x-aayaa", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 10, 3);
assertEquals("-x--yaa", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 10, 4);
assertEquals("-x--y-", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 10, 5);
assertEquals("-x--y-", sb.toString());
}
//-----------------------------------------------------------------------
public void testReverse() {
StrBuilder sb = new StrBuilder();
assertEquals("", sb.reverse().toString());
sb.clear().append(true);
assertEquals("eurt", sb.reverse().toString());
assertEquals("true", sb.reverse().toString());
}
//-----------------------------------------------------------------------
public void testTrim() {
StrBuilder sb = new StrBuilder();
assertEquals("", sb.reverse().toString());
sb.clear().append(" \u0000 ");
assertEquals("", sb.trim().toString());
sb.clear().append(" \u0000 a b c");
assertEquals("a b c", sb.trim().toString());
sb.clear().append("a b c \u0000 ");
assertEquals("a b c", sb.trim().toString());
sb.clear().append(" \u0000 a b c \u0000 ");
assertEquals("a b c", sb.trim().toString());
sb.clear().append("a b c");
assertEquals("a b c", sb.trim().toString());
}
//-----------------------------------------------------------------------
public void testStartsWith() {
StrBuilder sb = new StrBuilder();
assertFalse(sb.startsWith("a"));
assertFalse(sb.startsWith(null));
assertTrue(sb.startsWith(""));
sb.append("abc");
assertTrue(sb.startsWith("a"));
assertTrue(sb.startsWith("ab"));
assertTrue(sb.startsWith("abc"));
assertFalse(sb.startsWith("cba"));
}
public void testEndsWith() {
StrBuilder sb = new StrBuilder();
assertFalse(sb.endsWith("a"));
assertFalse(sb.endsWith("c"));
assertTrue(sb.endsWith(""));
assertFalse(sb.endsWith(null));
sb.append("abc");
assertTrue(sb.endsWith("c"));
assertTrue(sb.endsWith("bc"));
assertTrue(sb.endsWith("abc"));
assertFalse(sb.endsWith("cba"));
assertFalse(sb.endsWith("abcd"));
assertFalse(sb.endsWith(" abc"));
assertFalse(sb.endsWith("abc "));
}
//-----------------------------------------------------------------------
public void testSubstringInt() {
StrBuilder sb = new StrBuilder ("hello goodbye");
assertEquals ("goodbye", sb.substring(6));
assertEquals ("hello goodbye".substring(6), sb.substring(6));
assertEquals ("hello goodbye", sb.substring(0));
assertEquals ("hello goodbye".substring(0), sb.substring(0));
try {
sb.substring(-1);
fail ();
} catch (IndexOutOfBoundsException e) {}
try {
sb.substring(15);
fail ();
} catch (IndexOutOfBoundsException e) {}
}
public void testSubstringIntInt() {
StrBuilder sb = new StrBuilder ("hello goodbye");
assertEquals ("hello", sb.substring(0, 5));
assertEquals ("hello goodbye".substring(0, 6), sb.substring(0, 6));
assertEquals ("goodbye", sb.substring(6, 13));
assertEquals ("hello goodbye".substring(6,13), sb.substring(6, 13));
assertEquals ("goodbye", sb.substring(6, 20));
try {
sb.substring(-1, 5);
fail();
} catch (IndexOutOfBoundsException e) {}
try {
sb.substring(15, 20);
fail();
} catch (IndexOutOfBoundsException e) {}
}
// -----------------------------------------------------------------------
public void testMidString() {
StrBuilder sb = new StrBuilder("hello goodbye hello");
assertEquals("goodbye", sb.midString(6, 7));
assertEquals("hello", sb.midString(0, 5));
assertEquals("hello", sb.midString(-5, 5));
assertEquals("", sb.midString(0, -1));
assertEquals("", sb.midString(20, 2));
assertEquals("hello", sb.midString(14, 22));
}
public void testRightString() {
StrBuilder sb = new StrBuilder("left right");
assertEquals("right", sb.rightString(5));
assertEquals("", sb.rightString(0));
assertEquals("", sb.rightString(-5));
assertEquals("left right", sb.rightString(15));
}
public void testLeftString() {
StrBuilder sb = new StrBuilder("left right");
assertEquals("left", sb.leftString(4));
assertEquals("", sb.leftString(0));
assertEquals("", sb.leftString(-5));
assertEquals("left right", sb.leftString(15));
}
// -----------------------------------------------------------------------
public void testContains_char() {
StrBuilder sb = new StrBuilder("abcdefghijklmnopqrstuvwxyz");
assertEquals(true, sb.contains('a'));
assertEquals(true, sb.contains('o'));
assertEquals(true, sb.contains('z'));
assertEquals(false, sb.contains('1'));
}
public void testContains_String() {
StrBuilder sb = new StrBuilder("abcdefghijklmnopqrstuvwxyz");
assertEquals(true, sb.contains("a"));
assertEquals(true, sb.contains("pq"));
assertEquals(true, sb.contains("z"));
assertEquals(false, sb.contains("zyx"));
assertEquals(false, sb.contains((String) null));
}
public void testContains_StrMatcher() {
StrBuilder sb = new StrBuilder("abcdefghijklmnopqrstuvwxyz");
assertEquals(true, sb.contains(StrMatcher.charMatcher('a')));
assertEquals(true, sb.contains(StrMatcher.stringMatcher("pq")));
assertEquals(true, sb.contains(StrMatcher.charMatcher('z')));
assertEquals(false, sb.contains(StrMatcher.stringMatcher("zy")));
assertEquals(false, sb.contains((StrMatcher) null));
sb = new StrBuilder();
assertEquals(false, sb.contains(A_NUMBER_MATCHER));
sb.append("B A1 C");
assertEquals(true, sb.contains(A_NUMBER_MATCHER));
}
// -----------------------------------------------------------------------
public void testIndexOf_char() {
StrBuilder sb = new StrBuilder("abab");
assertEquals(0, sb.indexOf('a'));
// should work like String#indexOf
assertEquals("abab".indexOf('a'), sb.indexOf('a'));
assertEquals(1, sb.indexOf('b'));
assertEquals("abab".indexOf('b'), sb.indexOf('b'));
assertEquals(-1, sb.indexOf('z'));
}
public void testIndexOf_char_int() {
StrBuilder sb = new StrBuilder("abab");
assertEquals(0, sb.indexOf('a', -1));
assertEquals(0, sb.indexOf('a', 0));
assertEquals(2, sb.indexOf('a', 1));
assertEquals(-1, sb.indexOf('a', 4));
assertEquals(-1, sb.indexOf('a', 5));
// should work like String#indexOf
assertEquals("abab".indexOf('a', 1), sb.indexOf('a', 1));
assertEquals(3, sb.indexOf('b', 2));
assertEquals("abab".indexOf('b', 2), sb.indexOf('b', 2));
assertEquals(-1, sb.indexOf('z', 2));
sb = new StrBuilder("xyzabc");
assertEquals(2, sb.indexOf('z', 0));
assertEquals(-1, sb.indexOf('z', 3));
}
public void testLastIndexOf_char() {
StrBuilder sb = new StrBuilder("abab");
assertEquals (2, sb.lastIndexOf('a'));
//should work like String#lastIndexOf
assertEquals ("abab".lastIndexOf('a'), sb.lastIndexOf('a'));
assertEquals(3, sb.lastIndexOf('b'));
assertEquals ("abab".lastIndexOf('b'), sb.lastIndexOf('b'));
assertEquals (-1, sb.lastIndexOf('z'));
}
public void testLastIndexOf_char_int() {
StrBuilder sb = new StrBuilder("abab");
assertEquals(-1, sb.lastIndexOf('a', -1));
assertEquals(0, sb.lastIndexOf('a', 0));
assertEquals(0, sb.lastIndexOf('a', 1));
// should work like String#lastIndexOf
assertEquals("abab".lastIndexOf('a', 1), sb.lastIndexOf('a', 1));
assertEquals(1, sb.lastIndexOf('b', 2));
assertEquals("abab".lastIndexOf('b', 2), sb.lastIndexOf('b', 2));
assertEquals(-1, sb.lastIndexOf('z', 2));
sb = new StrBuilder("xyzabc");
assertEquals(2, sb.lastIndexOf('z', sb.length()));
assertEquals(-1, sb.lastIndexOf('z', 1));
}
// -----------------------------------------------------------------------
public void testIndexOf_String() {
StrBuilder sb = new StrBuilder("abab");
assertEquals(0, sb.indexOf("a"));
//should work like String#indexOf
assertEquals("abab".indexOf("a"), sb.indexOf("a"));
assertEquals(0, sb.indexOf("ab"));
//should work like String#indexOf
assertEquals("abab".indexOf("ab"), sb.indexOf("ab"));
assertEquals(1, sb.indexOf("b"));
assertEquals("abab".indexOf("b"), sb.indexOf("b"));
assertEquals(1, sb.indexOf("ba"));
assertEquals("abab".indexOf("ba"), sb.indexOf("ba"));
assertEquals(-1, sb.indexOf("z"));
assertEquals(-1, sb.indexOf((String) null));
}
public void testIndexOf_String_int() {
StrBuilder sb = new StrBuilder("abab");
assertEquals(0, sb.indexOf("a", -1));
assertEquals(0, sb.indexOf("a", 0));
assertEquals(2, sb.indexOf("a", 1));
assertEquals(2, sb.indexOf("a", 2));
assertEquals(-1, sb.indexOf("a", 3));
assertEquals(-1, sb.indexOf("a", 4));
assertEquals(-1, sb.indexOf("a", 5));
assertEquals(-1, sb.indexOf("abcdef", 0));
assertEquals(0, sb.indexOf("", 0));
assertEquals(1, sb.indexOf("", 1));
//should work like String#indexOf
assertEquals ("abab".indexOf("a", 1), sb.indexOf("a", 1));
assertEquals(2, sb.indexOf("ab", 1));
//should work like String#indexOf
assertEquals("abab".indexOf("ab", 1), sb.indexOf("ab", 1));
assertEquals(3, sb.indexOf("b", 2));
assertEquals("abab".indexOf("b", 2), sb.indexOf("b", 2));
assertEquals(1, sb.indexOf("ba", 1));
assertEquals("abab".indexOf("ba", 2), sb.indexOf("ba", 2));
assertEquals(-1, sb.indexOf("z", 2));
sb = new StrBuilder("xyzabc");
assertEquals(2, sb.indexOf("za", 0));
assertEquals(-1, sb.indexOf("za", 3));
assertEquals(-1, sb.indexOf((String) null, 2));
}
public void testLastIndexOf_String() {
StrBuilder sb = new StrBuilder("abab");
assertEquals(2, sb.lastIndexOf("a"));
//should work like String#lastIndexOf
assertEquals("abab".lastIndexOf("a"), sb.lastIndexOf("a"));
assertEquals(2, sb.lastIndexOf("ab"));
//should work like String#lastIndexOf
assertEquals("abab".lastIndexOf("ab"), sb.lastIndexOf("ab"));
assertEquals(3, sb.lastIndexOf("b"));
assertEquals("abab".lastIndexOf("b"), sb.lastIndexOf("b"));
assertEquals(1, sb.lastIndexOf("ba"));
assertEquals("abab".lastIndexOf("ba"), sb.lastIndexOf("ba"));
assertEquals(-1, sb.lastIndexOf("z"));
assertEquals(-1, sb.lastIndexOf((String) null));
}
public void testLastIndexOf_String_int() {
StrBuilder sb = new StrBuilder("abab");
assertEquals(-1, sb.lastIndexOf("a", -1));
assertEquals(0, sb.lastIndexOf("a", 0));
assertEquals(0, sb.lastIndexOf("a", 1));
assertEquals(2, sb.lastIndexOf("a", 2));
assertEquals(2, sb.lastIndexOf("a", 3));
assertEquals(2, sb.lastIndexOf("a", 4));
assertEquals(2, sb.lastIndexOf("a", 5));
assertEquals(-1, sb.lastIndexOf("abcdef", 3));
assertEquals("abab".lastIndexOf("", 3), sb.lastIndexOf("", 3));
assertEquals("abab".lastIndexOf("", 1), sb.lastIndexOf("", 1));
//should work like String#lastIndexOf
assertEquals("abab".lastIndexOf("a", 1), sb.lastIndexOf("a", 1));
assertEquals(0, sb.lastIndexOf("ab", 1));
//should work like String#lastIndexOf
assertEquals("abab".lastIndexOf("ab", 1), sb.lastIndexOf("ab", 1));
assertEquals(1, sb.lastIndexOf("b", 2));
assertEquals("abab".lastIndexOf("b", 2), sb.lastIndexOf("b", 2));
assertEquals(1, sb.lastIndexOf("ba", 2));
assertEquals("abab".lastIndexOf("ba", 2), sb.lastIndexOf("ba", 2));
assertEquals(-1, sb.lastIndexOf("z", 2));
sb = new StrBuilder("xyzabc");
assertEquals(2, sb.lastIndexOf("za", sb.length()));
assertEquals(-1, sb.lastIndexOf("za", 1));
assertEquals(-1, sb.lastIndexOf((String) null, 2));
}
// -----------------------------------------------------------------------
public void testIndexOf_StrMatcher() {
StrBuilder sb = new StrBuilder();
assertEquals(-1, sb.indexOf((StrMatcher) null));
assertEquals(-1, sb.indexOf(StrMatcher.charMatcher('a')));
sb.append("ab bd");
assertEquals(0, sb.indexOf(StrMatcher.charMatcher('a')));
assertEquals(1, sb.indexOf(StrMatcher.charMatcher('b')));
assertEquals(2, sb.indexOf(StrMatcher.spaceMatcher()));
assertEquals(4, sb.indexOf(StrMatcher.charMatcher('d')));
assertEquals(-1, sb.indexOf(StrMatcher.noneMatcher()));
assertEquals(-1, sb.indexOf((StrMatcher) null));
sb.append(" A1 junction");
assertEquals(6, sb.indexOf(A_NUMBER_MATCHER));
}
public void testIndexOf_StrMatcher_int() {
StrBuilder sb = new StrBuilder();
assertEquals(-1, sb.indexOf((StrMatcher) null, 2));
assertEquals(-1, sb.indexOf(StrMatcher.charMatcher('a'), 2));
assertEquals(-1, sb.indexOf(StrMatcher.charMatcher('a'), 0));
sb.append("ab bd");
assertEquals(0, sb.indexOf(StrMatcher.charMatcher('a'), -2));
assertEquals(0, sb.indexOf(StrMatcher.charMatcher('a'), 0));
assertEquals(-1, sb.indexOf(StrMatcher.charMatcher('a'), 2));
assertEquals(-1, sb.indexOf(StrMatcher.charMatcher('a'), 20));
assertEquals(1, sb.indexOf(StrMatcher.charMatcher('b'), -1));
assertEquals(1, sb.indexOf(StrMatcher.charMatcher('b'), 0));
assertEquals(1, sb.indexOf(StrMatcher.charMatcher('b'), 1));
assertEquals(3, sb.indexOf(StrMatcher.charMatcher('b'), 2));
assertEquals(3, sb.indexOf(StrMatcher.charMatcher('b'), 3));
assertEquals(-1, sb.indexOf(StrMatcher.charMatcher('b'), 4));
assertEquals(-1, sb.indexOf(StrMatcher.charMatcher('b'), 5));
assertEquals(-1, sb.indexOf(StrMatcher.charMatcher('b'), 6));
assertEquals(2, sb.indexOf(StrMatcher.spaceMatcher(), -2));
assertEquals(2, sb.indexOf(StrMatcher.spaceMatcher(), 0));
assertEquals(2, sb.indexOf(StrMatcher.spaceMatcher(), 2));
assertEquals(-1, sb.indexOf(StrMatcher.spaceMatcher(), 4));
assertEquals(-1, sb.indexOf(StrMatcher.spaceMatcher(), 20));
assertEquals(-1, sb.indexOf(StrMatcher.noneMatcher(), 0));
assertEquals(-1, sb.indexOf((StrMatcher) null, 0));
sb.append(" A1 junction with A2");
assertEquals(6, sb.indexOf(A_NUMBER_MATCHER, 5));
assertEquals(6, sb.indexOf(A_NUMBER_MATCHER, 6));
assertEquals(23, sb.indexOf(A_NUMBER_MATCHER, 7));
assertEquals(23, sb.indexOf(A_NUMBER_MATCHER, 22));
assertEquals(23, sb.indexOf(A_NUMBER_MATCHER, 23));
assertEquals(-1, sb.indexOf(A_NUMBER_MATCHER, 24));
}
public void testLastIndexOf_StrMatcher() {
StrBuilder sb = new StrBuilder();
assertEquals(-1, sb.lastIndexOf((StrMatcher) null));
assertEquals(-1, sb.lastIndexOf(StrMatcher.charMatcher('a')));
sb.append("ab bd");
assertEquals(0, sb.lastIndexOf(StrMatcher.charMatcher('a')));
assertEquals(3, sb.lastIndexOf(StrMatcher.charMatcher('b')));
assertEquals(2, sb.lastIndexOf(StrMatcher.spaceMatcher()));
assertEquals(4, sb.lastIndexOf(StrMatcher.charMatcher('d')));
assertEquals(-1, sb.lastIndexOf(StrMatcher.noneMatcher()));
assertEquals(-1, sb.lastIndexOf((StrMatcher) null));
sb.append(" A1 junction");
assertEquals(6, sb.lastIndexOf(A_NUMBER_MATCHER));
}
public void testLastIndexOf_StrMatcher_int() {
StrBuilder sb = new StrBuilder();
assertEquals(-1, sb.lastIndexOf((StrMatcher) null, 2));
assertEquals(-1, sb.lastIndexOf(StrMatcher.charMatcher('a'), 2));
assertEquals(-1, sb.lastIndexOf(StrMatcher.charMatcher('a'), 0));
assertEquals(-1, sb.lastIndexOf(StrMatcher.charMatcher('a'), -1));
sb.append("ab bd");
assertEquals(-1, sb.lastIndexOf(StrMatcher.charMatcher('a'), -2));
assertEquals(0, sb.lastIndexOf(StrMatcher.charMatcher('a'), 0));
assertEquals(0, sb.lastIndexOf(StrMatcher.charMatcher('a'), 2));
assertEquals(0, sb.lastIndexOf(StrMatcher.charMatcher('a'), 20));
assertEquals(-1, sb.lastIndexOf(StrMatcher.charMatcher('b'), -1));
assertEquals(-1, sb.lastIndexOf(StrMatcher.charMatcher('b'), 0));
assertEquals(1, sb.lastIndexOf(StrMatcher.charMatcher('b'), 1));
assertEquals(1, sb.lastIndexOf(StrMatcher.charMatcher('b'), 2));
assertEquals(3, sb.lastIndexOf(StrMatcher.charMatcher('b'), 3));
assertEquals(3, sb.lastIndexOf(StrMatcher.charMatcher('b'), 4));
assertEquals(3, sb.lastIndexOf(StrMatcher.charMatcher('b'), 5));
assertEquals(3, sb.lastIndexOf(StrMatcher.charMatcher('b'), 6));
assertEquals(-1, sb.lastIndexOf(StrMatcher.spaceMatcher(), -2));
assertEquals(-1, sb.lastIndexOf(StrMatcher.spaceMatcher(), 0));
assertEquals(2, sb.lastIndexOf(StrMatcher.spaceMatcher(), 2));
assertEquals(2, sb.lastIndexOf(StrMatcher.spaceMatcher(), 4));
assertEquals(2, sb.lastIndexOf(StrMatcher.spaceMatcher(), 20));
assertEquals(-1, sb.lastIndexOf(StrMatcher.noneMatcher(), 0));
assertEquals(-1, sb.lastIndexOf((StrMatcher) null, 0));
sb.append(" A1 junction with A2");
assertEquals(-1, sb.lastIndexOf(A_NUMBER_MATCHER, 5));
assertEquals(-1, sb.lastIndexOf(A_NUMBER_MATCHER, 6)); // A matches, 1 is outside bounds
assertEquals(6, sb.lastIndexOf(A_NUMBER_MATCHER, 7));
assertEquals(6, sb.lastIndexOf(A_NUMBER_MATCHER, 22));
assertEquals(6, sb.lastIndexOf(A_NUMBER_MATCHER, 23)); // A matches, 2 is outside bounds
assertEquals(23, sb.lastIndexOf(A_NUMBER_MATCHER, 24));
}
static final StrMatcher A_NUMBER_MATCHER = new StrMatcher() {
public int isMatch(char[] buffer, int pos, int bufferStart, int bufferEnd) {
if (buffer[pos] == 'A') {
pos++;
if (pos < bufferEnd && buffer[pos] >= '0' && buffer[pos] <= '9') {
return 2;
}
}
return 0;
}
};
//-----------------------------------------------------------------------
public void testAsTokenizer() throws Exception {
// from Javadoc
StrBuilder b = new StrBuilder();
b.append("a b ");
StrTokenizer t = b.asTokenizer();
String[] tokens1 = t.getTokenArray();
assertEquals(2, tokens1.length);
assertEquals("a", tokens1[0]);
assertEquals("b", tokens1[1]);
assertEquals(2, t.size());
b.append("c d ");
String[] tokens2 = t.getTokenArray();
assertEquals(2, tokens2.length);
assertEquals("a", tokens2[0]);
assertEquals("b", tokens2[1]);
assertEquals(2, t.size());
assertEquals("a", t.next());
assertEquals("b", t.next());
t.reset();
String[] tokens3 = t.getTokenArray();
assertEquals(4, tokens3.length);
assertEquals("a", tokens3[0]);
assertEquals("b", tokens3[1]);
assertEquals("c", tokens3[2]);
assertEquals("d", tokens3[3]);
assertEquals(4, t.size());
assertEquals("a", t.next());
assertEquals("b", t.next());
assertEquals("c", t.next());
assertEquals("d", t.next());
assertEquals("a b c d ", t.getContent());
}
// -----------------------------------------------------------------------
public void testAsReader() throws Exception {
StrBuilder sb = new StrBuilder("some text");
Reader reader = sb.asReader();
assertEquals(true, reader.ready());
char[] buf = new char[40];
assertEquals(9, reader.read(buf));
assertEquals("some text", new String(buf, 0, 9));
assertEquals(-1, reader.read());
assertEquals(false, reader.ready());
assertEquals(0, reader.skip(2));
assertEquals(0, reader.skip(-1));
assertEquals(true, reader.markSupported());
reader = sb.asReader();
assertEquals('s', reader.read());
reader.mark(-1);
char[] array = new char[3];
assertEquals(3, reader.read(array, 0, 3));
assertEquals('o', array[0]);
assertEquals('m', array[1]);
assertEquals('e', array[2]);
reader.reset();
assertEquals(1, reader.read(array, 1, 1));
assertEquals('o', array[0]);
assertEquals('o', array[1]);
assertEquals('e', array[2]);
assertEquals(2, reader.skip(2));
assertEquals(' ', reader.read());
assertEquals(true, reader.ready());
reader.close();
assertEquals(true, reader.ready());
reader = sb.asReader();
array = new char[3];
try {
reader.read(array, -1, 0);
fail();
} catch (IndexOutOfBoundsException ex) {}
try {
reader.read(array, 0, -1);
fail();
} catch (IndexOutOfBoundsException ex) {}
try {
reader.read(array, 100, 1);
fail();
} catch (IndexOutOfBoundsException ex) {}
try {
reader.read(array, 0, 100);
fail();
} catch (IndexOutOfBoundsException ex) {}
try {
reader.read(array, Integer.MAX_VALUE, Integer.MAX_VALUE);
fail();
} catch (IndexOutOfBoundsException ex) {}
assertEquals(0, reader.read(array, 0, 0));
assertEquals(0, array[0]);
assertEquals(0, array[1]);
assertEquals(0, array[2]);
reader.skip(9);
assertEquals(-1, reader.read(array, 0, 1));
reader.reset();
array = new char[30];
assertEquals(9, reader.read(array, 0, 30));
}
//-----------------------------------------------------------------------
public void testAsWriter() throws Exception {
StrBuilder sb = new StrBuilder("base");
Writer writer = sb.asWriter();
writer.write('l');
assertEquals("basel", sb.toString());
writer.write(new char[] {'i', 'n'});
assertEquals("baselin", sb.toString());
writer.write(new char[] {'n', 'e', 'r'}, 1, 2);
assertEquals("baseliner", sb.toString());
writer.write(" rout");
assertEquals("baseliner rout", sb.toString());
writer.write("ping that server", 1, 3);
assertEquals("baseliner routing", sb.toString());
writer.flush(); // no effect
assertEquals("baseliner routing", sb.toString());
writer.close(); // no effect
assertEquals("baseliner routing", sb.toString());
writer.write(" hi"); // works after close
assertEquals("baseliner routing hi", sb.toString());
sb.setLength(4); // mix and match
writer.write('d');
assertEquals("based", sb.toString());
}
//-----------------------------------------------------------------------
public void testEqualsIgnoreCase() {
StrBuilder sb1 = new StrBuilder();
StrBuilder sb2 = new StrBuilder();
assertEquals(true, sb1.equalsIgnoreCase(sb1));
assertEquals(true, sb1.equalsIgnoreCase(sb2));
assertEquals(true, sb2.equalsIgnoreCase(sb2));
sb1.append("abc");
assertEquals(false, sb1.equalsIgnoreCase(sb2));
sb2.append("ABC");
assertEquals(true, sb1.equalsIgnoreCase(sb2));
sb2.clear().append("abc");
assertEquals(true, sb1.equalsIgnoreCase(sb2));
assertEquals(true, sb1.equalsIgnoreCase(sb1));
assertEquals(true, sb2.equalsIgnoreCase(sb2));
sb2.clear().append("aBc");
assertEquals(true, sb1.equalsIgnoreCase(sb2));
}
//-----------------------------------------------------------------------
public void testEquals() {
StrBuilder sb1 = new StrBuilder();
StrBuilder sb2 = new StrBuilder();
assertEquals(true, sb1.equals(sb2));
assertEquals(true, sb1.equals(sb1));
assertEquals(true, sb2.equals(sb2));
assertEquals(true, sb1.equals((Object) sb2));
sb1.append("abc");
assertEquals(false, sb1.equals(sb2));
assertEquals(false, sb1.equals((Object) sb2));
sb2.append("ABC");
assertEquals(false, sb1.equals(sb2));
assertEquals(false, sb1.equals((Object) sb2));
sb2.clear().append("abc");
assertEquals(true, sb1.equals(sb2));
assertEquals(true, sb1.equals((Object) sb2));
assertEquals(false, sb1.equals(new Integer(1)));
assertEquals(false, sb1.equals("abc"));
}
//-----------------------------------------------------------------------
public void testHashCode() {
StrBuilder sb = new StrBuilder();
int hc1a = sb.hashCode();
int hc1b = sb.hashCode();
assertEquals(0, hc1a);
assertEquals(hc1a, hc1b);
sb.append("abc");
int hc2a = sb.hashCode();
int hc2b = sb.hashCode();
assertEquals(true, hc2a != 0);
assertEquals(hc2a, hc2b);
}
//-----------------------------------------------------------------------
public void testToString() {
StrBuilder sb = new StrBuilder("abc");
assertEquals("abc", sb.toString());
}
//-----------------------------------------------------------------------
public void testToStringBuffer() {
StrBuilder sb = new StrBuilder();
assertEquals(new StringBuffer().toString(), sb.toStringBuffer().toString());
sb.append("junit");
assertEquals(new StringBuffer("junit").toString(), sb.toStringBuffer().toString());
}
//-----------------------------------------------------------------------
public void testLang294() {
StrBuilder sb = new StrBuilder("\n%BLAH%\nDo more stuff\neven more stuff\n%BLAH%\n");
sb.deleteAll("\n%BLAH%");
assertEquals("\nDo more stuff\neven more stuff\n", sb.toString());
}
public void testIndexOfLang294() {
StrBuilder sb = new StrBuilder("onetwothree");
sb.deleteFirst("three");
assertEquals(-1, sb.indexOf("three"));
}
} | // You are a professional Java test case writer, please create a test case named `testIndexOfLang294` for the issue `Lang-LANG-294`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Lang-LANG-294
//
// ## Issue-Title:
// StrBuilder.replaceAll and StrBuilder.deleteAll can throw ArrayIndexOutOfBoundsException.
//
// ## Issue-Description:
//
// StrBuilder.replaceAll and StrBuilder.deleteAll can thrown ArrayIndexOutOfBoundsException's. Here are a couple of additions to the StrBuilderTest class that demonstrate this problem:
//
//
// StrBuilder.deleteAll() - added to testDeleteAll\_String():
//
//
// sb = new StrBuilder("\n%BLAH%\nDo more stuff\neven more stuff\n%BLAH%\n");
//
// sb.deleteAll("\n%BLAH%");
//
// assertEquals("\nDo more stuff\neven more stuff\n", sb.toString());
//
//
// this causes the following error:
//
// java.lang.ArrayIndexOutOfBoundsException
//
// at java.lang.System.arraycopy(Native Method)
//
// at org.apache.commons.lang.text.StrBuilder.deleteImpl(StrBuilder.java:1114)
//
// at org.apache.commons.lang.text.StrBuilder.deleteAll(StrBuilder.java:1188)
//
// at org.apache.commons.lang.text.StrBuilderTest.testDeleteAll\_String(StrBuilderTest.java:606)
//
// at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
//
// at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:39)
//
// at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:25)
//
// at java.lang.reflect.Method.invoke(Method.java:585)
//
// at junit.framework.TestCase.runTest(TestCase.java:154)
//
// at junit.framework.TestCase.runBare(TestCase.java:127)
//
// at junit.framework.TestResult$1.protect(TestResult.java:106)
//
// at junit.framework.TestResult.runProtected(TestResult.java:124)
//
// at junit.framework.TestResult.run(TestResult.java:109)
//
// at junit.framework.TestCase.run(TestCase.java:118)
//
// at junit.framework.TestSuite.runTest(TestSuite.java:208)
//
// at junit.framework.TestSuite.run(TestSuite.java:203)
//
// at org.eclipse.jdt.internal.junit.runner.junit3.JUnit3TestReference.run(JUnit3TestReference.java:128)
//
// at org.eclipse.jdt.internal.junit.runner.TestExecution.run(TestExecution.java:38)
//
// at org.eclipse.jdt.internal.junit.runner.RemoteTestRunner.runTests(RemoteTestRunner.java:460)
//
// at org.eclipse.jdt.internal.junit.runner.RemoteTestRunner.runTests(RemoteTestRunner.java:673)
//
// at org.eclipse.jdt.internal.junit.runner.RemoteTestRunner.run(RemoteTestRunner.java:386)
//
// at org.eclipse.jdt.internal.junit.runner.RemoteTestRunner.main(RemoteTestRunner.java:196)
//
//
// StrBuilder.replaceAll() - added to testReplaceAll\_String\_String():
//
//
// sb = new StrBuilder("\n%BLAH%\nDo more stuff\neven more stuff\n%BLAH%\n");
//
// sb.replaceAll("\n%BLAH%", "");
//
// assertEquals("\nDo more stuff\neven more stuff\n", sb.toString());
//
//
// this causes the exception:
//
//
// java.lang.ArrayIndexOutOfBoundsException
//
// at java.lang.System.arraycopy(Native Method)
//
// at org.apache.commons.lang.text.StrBuilder.replaceImpl(StrBuilder.java:1256)
//
// at org.apache.commons.lang.text.StrBuilder.replaceAll(StrBuilder.java:1339)
//
// at org.apache.commons.lang.text.StrBuilderTest.testReplaceAll\_String\_String
public void testIndexOfLang294() {
| 1,741 | 61 | 1,737 | src/test/org/apache/commons/lang/text/StrBuilderTest.java | src/test | ```markdown
## Issue-ID: Lang-LANG-294
## Issue-Title:
StrBuilder.replaceAll and StrBuilder.deleteAll can throw ArrayIndexOutOfBoundsException.
## Issue-Description:
StrBuilder.replaceAll and StrBuilder.deleteAll can thrown ArrayIndexOutOfBoundsException's. Here are a couple of additions to the StrBuilderTest class that demonstrate this problem:
StrBuilder.deleteAll() - added to testDeleteAll\_String():
sb = new StrBuilder("\n%BLAH%\nDo more stuff\neven more stuff\n%BLAH%\n");
sb.deleteAll("\n%BLAH%");
assertEquals("\nDo more stuff\neven more stuff\n", sb.toString());
this causes the following error:
java.lang.ArrayIndexOutOfBoundsException
at java.lang.System.arraycopy(Native Method)
at org.apache.commons.lang.text.StrBuilder.deleteImpl(StrBuilder.java:1114)
at org.apache.commons.lang.text.StrBuilder.deleteAll(StrBuilder.java:1188)
at org.apache.commons.lang.text.StrBuilderTest.testDeleteAll\_String(StrBuilderTest.java:606)
at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:39)
at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:25)
at java.lang.reflect.Method.invoke(Method.java:585)
at junit.framework.TestCase.runTest(TestCase.java:154)
at junit.framework.TestCase.runBare(TestCase.java:127)
at junit.framework.TestResult$1.protect(TestResult.java:106)
at junit.framework.TestResult.runProtected(TestResult.java:124)
at junit.framework.TestResult.run(TestResult.java:109)
at junit.framework.TestCase.run(TestCase.java:118)
at junit.framework.TestSuite.runTest(TestSuite.java:208)
at junit.framework.TestSuite.run(TestSuite.java:203)
at org.eclipse.jdt.internal.junit.runner.junit3.JUnit3TestReference.run(JUnit3TestReference.java:128)
at org.eclipse.jdt.internal.junit.runner.TestExecution.run(TestExecution.java:38)
at org.eclipse.jdt.internal.junit.runner.RemoteTestRunner.runTests(RemoteTestRunner.java:460)
at org.eclipse.jdt.internal.junit.runner.RemoteTestRunner.runTests(RemoteTestRunner.java:673)
at org.eclipse.jdt.internal.junit.runner.RemoteTestRunner.run(RemoteTestRunner.java:386)
at org.eclipse.jdt.internal.junit.runner.RemoteTestRunner.main(RemoteTestRunner.java:196)
StrBuilder.replaceAll() - added to testReplaceAll\_String\_String():
sb = new StrBuilder("\n%BLAH%\nDo more stuff\neven more stuff\n%BLAH%\n");
sb.replaceAll("\n%BLAH%", "");
assertEquals("\nDo more stuff\neven more stuff\n", sb.toString());
this causes the exception:
java.lang.ArrayIndexOutOfBoundsException
at java.lang.System.arraycopy(Native Method)
at org.apache.commons.lang.text.StrBuilder.replaceImpl(StrBuilder.java:1256)
at org.apache.commons.lang.text.StrBuilder.replaceAll(StrBuilder.java:1339)
at org.apache.commons.lang.text.StrBuilderTest.testReplaceAll\_String\_String
```
You are a professional Java test case writer, please create a test case named `testIndexOfLang294` for the issue `Lang-LANG-294`, utilizing the provided issue report information and the following function signature.
```java
public void testIndexOfLang294() {
```
| 1,737 | [
"org.apache.commons.lang.text.StrBuilder"
] | a61dc21c8d973ee2be051395440fe87ed0b2c5e6316fad61fc86e80358e561ea | public void testIndexOfLang294() | // You are a professional Java test case writer, please create a test case named `testIndexOfLang294` for the issue `Lang-LANG-294`, utilizing the provided issue report information and the following function signature.
// ## Issue-ID: Lang-LANG-294
//
// ## Issue-Title:
// StrBuilder.replaceAll and StrBuilder.deleteAll can throw ArrayIndexOutOfBoundsException.
//
// ## Issue-Description:
//
// StrBuilder.replaceAll and StrBuilder.deleteAll can thrown ArrayIndexOutOfBoundsException's. Here are a couple of additions to the StrBuilderTest class that demonstrate this problem:
//
//
// StrBuilder.deleteAll() - added to testDeleteAll\_String():
//
//
// sb = new StrBuilder("\n%BLAH%\nDo more stuff\neven more stuff\n%BLAH%\n");
//
// sb.deleteAll("\n%BLAH%");
//
// assertEquals("\nDo more stuff\neven more stuff\n", sb.toString());
//
//
// this causes the following error:
//
// java.lang.ArrayIndexOutOfBoundsException
//
// at java.lang.System.arraycopy(Native Method)
//
// at org.apache.commons.lang.text.StrBuilder.deleteImpl(StrBuilder.java:1114)
//
// at org.apache.commons.lang.text.StrBuilder.deleteAll(StrBuilder.java:1188)
//
// at org.apache.commons.lang.text.StrBuilderTest.testDeleteAll\_String(StrBuilderTest.java:606)
//
// at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
//
// at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:39)
//
// at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:25)
//
// at java.lang.reflect.Method.invoke(Method.java:585)
//
// at junit.framework.TestCase.runTest(TestCase.java:154)
//
// at junit.framework.TestCase.runBare(TestCase.java:127)
//
// at junit.framework.TestResult$1.protect(TestResult.java:106)
//
// at junit.framework.TestResult.runProtected(TestResult.java:124)
//
// at junit.framework.TestResult.run(TestResult.java:109)
//
// at junit.framework.TestCase.run(TestCase.java:118)
//
// at junit.framework.TestSuite.runTest(TestSuite.java:208)
//
// at junit.framework.TestSuite.run(TestSuite.java:203)
//
// at org.eclipse.jdt.internal.junit.runner.junit3.JUnit3TestReference.run(JUnit3TestReference.java:128)
//
// at org.eclipse.jdt.internal.junit.runner.TestExecution.run(TestExecution.java:38)
//
// at org.eclipse.jdt.internal.junit.runner.RemoteTestRunner.runTests(RemoteTestRunner.java:460)
//
// at org.eclipse.jdt.internal.junit.runner.RemoteTestRunner.runTests(RemoteTestRunner.java:673)
//
// at org.eclipse.jdt.internal.junit.runner.RemoteTestRunner.run(RemoteTestRunner.java:386)
//
// at org.eclipse.jdt.internal.junit.runner.RemoteTestRunner.main(RemoteTestRunner.java:196)
//
//
// StrBuilder.replaceAll() - added to testReplaceAll\_String\_String():
//
//
// sb = new StrBuilder("\n%BLAH%\nDo more stuff\neven more stuff\n%BLAH%\n");
//
// sb.replaceAll("\n%BLAH%", "");
//
// assertEquals("\nDo more stuff\neven more stuff\n", sb.toString());
//
//
// this causes the exception:
//
//
// java.lang.ArrayIndexOutOfBoundsException
//
// at java.lang.System.arraycopy(Native Method)
//
// at org.apache.commons.lang.text.StrBuilder.replaceImpl(StrBuilder.java:1256)
//
// at org.apache.commons.lang.text.StrBuilder.replaceAll(StrBuilder.java:1339)
//
// at org.apache.commons.lang.text.StrBuilderTest.testReplaceAll\_String\_String
| Lang | /*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.lang.text;
import java.io.Reader;
import java.io.Writer;
import java.util.Arrays;
import junit.framework.Test;
import junit.framework.TestCase;
import junit.framework.TestSuite;
import junit.textui.TestRunner;
import org.apache.commons.lang.ArrayUtils;
/**
* Unit tests for {@link org.apache.commons.lang.text.StrBuilder}.
*
* @author Michael Heuer
* @version $Id$
*/
public class StrBuilderTest extends TestCase {
/**
* Main method.
*
* @param args command line arguments, ignored
*/
public static void main(String[] args) {
TestRunner.run(suite());
}
/**
* Return a new test suite containing this test case.
*
* @return a new test suite containing this test case
*/
public static Test suite() {
TestSuite suite = new TestSuite(StrBuilderTest.class);
suite.setName("StrBuilder Tests");
return suite;
}
/**
* Create a new test case with the specified name.
*
* @param name
* name
*/
public StrBuilderTest(String name) {
super(name);
}
//-----------------------------------------------------------------------
public void testConstructors() {
StrBuilder sb0 = new StrBuilder();
assertEquals(32, sb0.capacity());
assertEquals(0, sb0.length());
assertEquals(0, sb0.size());
StrBuilder sb1 = new StrBuilder(32);
assertEquals(32, sb1.capacity());
assertEquals(0, sb1.length());
assertEquals(0, sb1.size());
StrBuilder sb2 = new StrBuilder(0);
assertEquals(32, sb2.capacity());
assertEquals(0, sb2.length());
assertEquals(0, sb2.size());
StrBuilder sb3 = new StrBuilder(-1);
assertEquals(32, sb3.capacity());
assertEquals(0, sb3.length());
assertEquals(0, sb3.size());
StrBuilder sb4 = new StrBuilder(1);
assertEquals(1, sb4.capacity());
assertEquals(0, sb4.length());
assertEquals(0, sb4.size());
StrBuilder sb5 = new StrBuilder((String) null);
assertEquals(32, sb5.capacity());
assertEquals(0, sb5.length());
assertEquals(0, sb5.size());
StrBuilder sb6 = new StrBuilder("");
assertEquals(32, sb6.capacity());
assertEquals(0, sb6.length());
assertEquals(0, sb6.size());
StrBuilder sb7 = new StrBuilder("foo");
assertEquals(35, sb7.capacity());
assertEquals(3, sb7.length());
assertEquals(3, sb7.size());
}
//-----------------------------------------------------------------------
public void testChaining() {
StrBuilder sb = new StrBuilder();
assertSame(sb, sb.setNewLineText(null));
assertSame(sb, sb.setNullText(null));
assertSame(sb, sb.setLength(1));
assertSame(sb, sb.setCharAt(0, 'a'));
assertSame(sb, sb.ensureCapacity(0));
assertSame(sb, sb.minimizeCapacity());
assertSame(sb, sb.clear());
assertSame(sb, sb.reverse());
assertSame(sb, sb.trim());
}
//-----------------------------------------------------------------------
public void testGetSetNewLineText() {
StrBuilder sb = new StrBuilder();
assertEquals(null, sb.getNewLineText());
sb.setNewLineText("#");
assertEquals("#", sb.getNewLineText());
sb.setNewLineText("");
assertEquals("", sb.getNewLineText());
sb.setNewLineText((String) null);
assertEquals(null, sb.getNewLineText());
}
//-----------------------------------------------------------------------
public void testGetSetNullText() {
StrBuilder sb = new StrBuilder();
assertEquals(null, sb.getNullText());
sb.setNullText("null");
assertEquals("null", sb.getNullText());
sb.setNullText("");
assertEquals(null, sb.getNullText());
sb.setNullText("NULL");
assertEquals("NULL", sb.getNullText());
sb.setNullText((String) null);
assertEquals(null, sb.getNullText());
}
//-----------------------------------------------------------------------
public void testCapacityAndLength() {
StrBuilder sb = new StrBuilder();
assertEquals(32, sb.capacity());
assertEquals(0, sb.length());
assertEquals(0, sb.size());
assertTrue(sb.isEmpty());
sb.minimizeCapacity();
assertEquals(0, sb.capacity());
assertEquals(0, sb.length());
assertEquals(0, sb.size());
assertTrue(sb.isEmpty());
sb.ensureCapacity(32);
assertTrue(sb.capacity() >= 32);
assertEquals(0, sb.length());
assertEquals(0, sb.size());
assertTrue(sb.isEmpty());
sb.append("foo");
assertTrue(sb.capacity() >= 32);
assertEquals(3, sb.length());
assertEquals(3, sb.size());
assertTrue(sb.isEmpty() == false);
sb.clear();
assertTrue(sb.capacity() >= 32);
assertEquals(0, sb.length());
assertEquals(0, sb.size());
assertTrue(sb.isEmpty());
sb.append("123456789012345678901234567890123");
assertTrue(sb.capacity() > 32);
assertEquals(33, sb.length());
assertEquals(33, sb.size());
assertTrue(sb.isEmpty() == false);
sb.ensureCapacity(16);
assertTrue(sb.capacity() > 16);
assertEquals(33, sb.length());
assertEquals(33, sb.size());
assertTrue(sb.isEmpty() == false);
sb.minimizeCapacity();
assertEquals(33, sb.capacity());
assertEquals(33, sb.length());
assertEquals(33, sb.size());
assertTrue(sb.isEmpty() == false);
try {
sb.setLength(-1);
fail("setLength(-1) expected StringIndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {
// expected
}
sb.setLength(33);
assertEquals(33, sb.capacity());
assertEquals(33, sb.length());
assertEquals(33, sb.size());
assertTrue(sb.isEmpty() == false);
sb.setLength(16);
assertTrue(sb.capacity() >= 16);
assertEquals(16, sb.length());
assertEquals(16, sb.size());
assertEquals("1234567890123456", sb.toString());
assertTrue(sb.isEmpty() == false);
sb.setLength(32);
assertTrue(sb.capacity() >= 32);
assertEquals(32, sb.length());
assertEquals(32, sb.size());
assertEquals("1234567890123456\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0", sb.toString());
assertTrue(sb.isEmpty() == false);
sb.setLength(0);
assertTrue(sb.capacity() >= 32);
assertEquals(0, sb.length());
assertEquals(0, sb.size());
assertTrue(sb.isEmpty());
}
//-----------------------------------------------------------------------
public void testLength() {
StrBuilder sb = new StrBuilder();
assertEquals(0, sb.length());
sb.append("Hello");
assertEquals(5, sb.length());
}
public void testSetLength() {
StrBuilder sb = new StrBuilder();
sb.append("Hello");
sb.setLength(2); // shorten
assertEquals("He", sb.toString());
sb.setLength(2); // no change
assertEquals("He", sb.toString());
sb.setLength(3); // lengthen
assertEquals("He\0", sb.toString());
try {
sb.setLength(-1);
fail("setLength(-1) expected StringIndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {
// expected
}
}
//-----------------------------------------------------------------------
public void testCapacity() {
StrBuilder sb = new StrBuilder();
assertEquals(sb.buffer.length, sb.capacity());
sb.append("HelloWorldHelloWorldHelloWorldHelloWorld");
assertEquals(sb.buffer.length, sb.capacity());
}
public void testEnsureCapacity() {
StrBuilder sb = new StrBuilder();
sb.ensureCapacity(2);
assertEquals(true, sb.capacity() >= 2);
sb.ensureCapacity(-1);
assertEquals(true, sb.capacity() >= 0);
sb.append("HelloWorld");
sb.ensureCapacity(40);
assertEquals(true, sb.capacity() >= 40);
}
public void testMinimizeCapacity() {
StrBuilder sb = new StrBuilder();
sb.minimizeCapacity();
assertEquals(0, sb.capacity());
sb.append("HelloWorld");
sb.minimizeCapacity();
assertEquals(10, sb.capacity());
}
//-----------------------------------------------------------------------
public void testSize() {
StrBuilder sb = new StrBuilder();
assertEquals(0, sb.size());
sb.append("Hello");
assertEquals(5, sb.size());
}
public void testIsEmpty() {
StrBuilder sb = new StrBuilder();
assertEquals(true, sb.isEmpty());
sb.append("Hello");
assertEquals(false, sb.isEmpty());
sb.clear();
assertEquals(true, sb.isEmpty());
}
public void testClear() {
StrBuilder sb = new StrBuilder();
sb.append("Hello");
sb.clear();
assertEquals(0, sb.length());
assertEquals(true, sb.buffer.length >= 5);
}
//-----------------------------------------------------------------------
public void testCharAt() {
StrBuilder sb = new StrBuilder();
try {
sb.charAt(0);
fail("charAt(0) expected IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {
// expected
}
try {
sb.charAt(-1);
fail("charAt(-1) expected IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {
// expected
}
sb.append("foo");
assertEquals('f', sb.charAt(0));
assertEquals('o', sb.charAt(1));
assertEquals('o', sb.charAt(2));
try {
sb.charAt(-1);
fail("charAt(-1) expected IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {
// expected
}
try {
sb.charAt(3);
fail("charAt(3) expected IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {
// expected
}
}
//-----------------------------------------------------------------------
public void testSetCharAt() {
StrBuilder sb = new StrBuilder();
try {
sb.setCharAt(0, 'f');
fail("setCharAt(0,) expected IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {
// expected
}
try {
sb.setCharAt(-1, 'f');
fail("setCharAt(-1,) expected IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {
// expected
}
sb.append("foo");
sb.setCharAt(0, 'b');
sb.setCharAt(1, 'a');
sb.setCharAt(2, 'r');
try {
sb.setCharAt(3, '!');
fail("setCharAt(3,) expected IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {
// expected
}
assertEquals("bar", sb.toString());
}
//-----------------------------------------------------------------------
public void testDeleteCharAt() {
StrBuilder sb = new StrBuilder("abc");
sb.deleteCharAt(0);
assertEquals("bc", sb.toString());
try {
sb.deleteCharAt(1000);
fail("Expected IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {}
}
//-----------------------------------------------------------------------
public void testToCharArray() {
StrBuilder sb = new StrBuilder();
assertEquals(ArrayUtils.EMPTY_CHAR_ARRAY, sb.toCharArray());
char[] a = sb.toCharArray();
assertNotNull("toCharArray() result is null", a);
assertEquals("toCharArray() result is too large", 0, a.length);
sb.append("junit");
a = sb.toCharArray();
assertEquals("toCharArray() result incorrect length", 5, a.length);
assertTrue("toCharArray() result does not match", Arrays.equals("junit".toCharArray(), a));
}
public void testToCharArrayIntInt() {
StrBuilder sb = new StrBuilder();
assertEquals(ArrayUtils.EMPTY_CHAR_ARRAY, sb.toCharArray(0, 0));
sb.append("junit");
char[] a = sb.toCharArray(0, 20); // too large test
assertEquals("toCharArray(int,int) result incorrect length", 5, a.length);
assertTrue("toCharArray(int,int) result does not match", Arrays.equals("junit".toCharArray(), a));
a = sb.toCharArray(0, 4);
assertEquals("toCharArray(int,int) result incorrect length", 4, a.length);
assertTrue("toCharArray(int,int) result does not match", Arrays.equals("juni".toCharArray(), a));
a = sb.toCharArray(0, 4);
assertEquals("toCharArray(int,int) result incorrect length", 4, a.length);
assertTrue("toCharArray(int,int) result does not match", Arrays.equals("juni".toCharArray(), a));
a = sb.toCharArray(0, 1);
assertNotNull("toCharArray(int,int) result is null", a);
try {
sb.toCharArray(-1, 5);
fail("no string index out of bound on -1");
} catch (IndexOutOfBoundsException e) {
}
try {
sb.toCharArray(6, 5);
fail("no string index out of bound on -1");
} catch (IndexOutOfBoundsException e) {
}
}
public void testGetChars ( ) {
StrBuilder sb = new StrBuilder();
char[] input = new char[10];
char[] a = sb.getChars(input);
assertSame (input, a);
assertTrue(Arrays.equals(new char[10], a));
sb.append("junit");
a = sb.getChars(input);
assertSame(input, a);
assertTrue(Arrays.equals(new char[] {'j','u','n','i','t',0,0,0,0,0},a));
a = sb.getChars(null);
assertNotSame(input,a);
assertEquals(5,a.length);
assertTrue(Arrays.equals("junit".toCharArray(),a));
input = new char[5];
a = sb.getChars(input);
assertSame(input, a);
input = new char[4];
a = sb.getChars(input);
assertNotSame(input, a);
}
public void testGetCharsIntIntCharArrayInt( ) {
StrBuilder sb = new StrBuilder();
sb.append("junit");
char[] a = new char[5];
sb.getChars(0,5,a,0);
assertTrue(Arrays.equals(new char[] {'j','u','n','i','t'},a));
a = new char[5];
sb.getChars(0,2,a,3);
assertTrue(Arrays.equals(new char[] {0,0,0,'j','u'},a));
try {
sb.getChars(-1,0,a,0);
fail("no exception");
}
catch (IndexOutOfBoundsException e) {
}
try {
sb.getChars(0,-1,a,0);
fail("no exception");
}
catch (IndexOutOfBoundsException e) {
}
try {
sb.getChars(0,20,a,0);
fail("no exception");
}
catch (IndexOutOfBoundsException e) {
}
try {
sb.getChars(4,2,a,0);
fail("no exception");
}
catch (IndexOutOfBoundsException e) {
}
}
//-----------------------------------------------------------------------
public void testDeleteIntInt() {
StrBuilder sb = new StrBuilder("abc");
sb.delete(0, 1);
assertEquals("bc", sb.toString());
sb.delete(1, 2);
assertEquals("b", sb.toString());
sb.delete(0, 1);
assertEquals("", sb.toString());
sb.delete(0, 1000);
assertEquals("", sb.toString());
try {
sb.delete(1, 2);
fail("Expected IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {}
try {
sb.delete(-1, 1);
fail("Expected IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {}
sb = new StrBuilder("anything");
try {
sb.delete(2, 1);
fail("Expected IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {}
}
//-----------------------------------------------------------------------
public void testDeleteAll_char() {
StrBuilder sb = new StrBuilder("abcbccba");
sb.deleteAll('X');
assertEquals("abcbccba", sb.toString());
sb.deleteAll('a');
assertEquals("bcbccb", sb.toString());
sb.deleteAll('c');
assertEquals("bbb", sb.toString());
sb.deleteAll('b');
assertEquals("", sb.toString());
sb = new StrBuilder("");
sb.deleteAll('b');
assertEquals("", sb.toString());
}
public void testDeleteFirst_char() {
StrBuilder sb = new StrBuilder("abcba");
sb.deleteFirst('X');
assertEquals("abcba", sb.toString());
sb.deleteFirst('a');
assertEquals("bcba", sb.toString());
sb.deleteFirst('c');
assertEquals("bba", sb.toString());
sb.deleteFirst('b');
assertEquals("ba", sb.toString());
sb = new StrBuilder("");
sb.deleteFirst('b');
assertEquals("", sb.toString());
}
// -----------------------------------------------------------------------
public void testDeleteAll_String() {
StrBuilder sb = new StrBuilder("abcbccba");
sb.deleteAll((String) null);
assertEquals("abcbccba", sb.toString());
sb.deleteAll("");
assertEquals("abcbccba", sb.toString());
sb.deleteAll("X");
assertEquals("abcbccba", sb.toString());
sb.deleteAll("a");
assertEquals("bcbccb", sb.toString());
sb.deleteAll("c");
assertEquals("bbb", sb.toString());
sb.deleteAll("b");
assertEquals("", sb.toString());
sb = new StrBuilder("abcbccba");
sb.deleteAll("bc");
assertEquals("acba", sb.toString());
sb = new StrBuilder("");
sb.deleteAll("bc");
assertEquals("", sb.toString());
}
public void testDeleteFirst_String() {
StrBuilder sb = new StrBuilder("abcbccba");
sb.deleteFirst((String) null);
assertEquals("abcbccba", sb.toString());
sb.deleteFirst("");
assertEquals("abcbccba", sb.toString());
sb.deleteFirst("X");
assertEquals("abcbccba", sb.toString());
sb.deleteFirst("a");
assertEquals("bcbccba", sb.toString());
sb.deleteFirst("c");
assertEquals("bbccba", sb.toString());
sb.deleteFirst("b");
assertEquals("bccba", sb.toString());
sb = new StrBuilder("abcbccba");
sb.deleteFirst("bc");
assertEquals("abccba", sb.toString());
sb = new StrBuilder("");
sb.deleteFirst("bc");
assertEquals("", sb.toString());
}
// -----------------------------------------------------------------------
public void testDeleteAll_StrMatcher() {
StrBuilder sb = new StrBuilder("A0xA1A2yA3");
sb.deleteAll((StrMatcher) null);
assertEquals("A0xA1A2yA3", sb.toString());
sb.deleteAll(A_NUMBER_MATCHER);
assertEquals("xy", sb.toString());
sb = new StrBuilder("Ax1");
sb.deleteAll(A_NUMBER_MATCHER);
assertEquals("Ax1", sb.toString());
sb = new StrBuilder("");
sb.deleteAll(A_NUMBER_MATCHER);
assertEquals("", sb.toString());
}
public void testDeleteFirst_StrMatcher() {
StrBuilder sb = new StrBuilder("A0xA1A2yA3");
sb.deleteFirst((StrMatcher) null);
assertEquals("A0xA1A2yA3", sb.toString());
sb.deleteFirst(A_NUMBER_MATCHER);
assertEquals("xA1A2yA3", sb.toString());
sb = new StrBuilder("Ax1");
sb.deleteFirst(A_NUMBER_MATCHER);
assertEquals("Ax1", sb.toString());
sb = new StrBuilder("");
sb.deleteFirst(A_NUMBER_MATCHER);
assertEquals("", sb.toString());
}
// -----------------------------------------------------------------------
public void testReplace_int_int_String() {
StrBuilder sb = new StrBuilder("abc");
sb.replace(0, 1, "d");
assertEquals("dbc", sb.toString());
sb.replace(0, 1, "aaa");
assertEquals("aaabc", sb.toString());
sb.replace(0, 3, "");
assertEquals("bc", sb.toString());
sb.replace(1, 2, (String) null);
assertEquals("b", sb.toString());
sb.replace(1, 1000, "text");
assertEquals("btext", sb.toString());
sb.replace(0, 1000, "text");
assertEquals("text", sb.toString());
sb = new StrBuilder("atext");
sb.replace(1, 1, "ny");
assertEquals("anytext", sb.toString());
try {
sb.replace(2, 1, "anything");
fail("Expected IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {}
sb = new StrBuilder();
try {
sb.replace(1, 2, "anything");
fail("Expected IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {}
try {
sb.replace(-1, 1, "anything");
fail("Expected IndexOutOfBoundsException");
} catch (IndexOutOfBoundsException e) {}
}
//-----------------------------------------------------------------------
public void testReplaceAll_char_char() {
StrBuilder sb = new StrBuilder("abcbccba");
sb.replaceAll('x', 'y');
assertEquals("abcbccba", sb.toString());
sb.replaceAll('a', 'd');
assertEquals("dbcbccbd", sb.toString());
sb.replaceAll('b', 'e');
assertEquals("dececced", sb.toString());
sb.replaceAll('c', 'f');
assertEquals("defeffed", sb.toString());
sb.replaceAll('d', 'd');
assertEquals("defeffed", sb.toString());
}
//-----------------------------------------------------------------------
public void testReplaceFirst_char_char() {
StrBuilder sb = new StrBuilder("abcbccba");
sb.replaceFirst('x', 'y');
assertEquals("abcbccba", sb.toString());
sb.replaceFirst('a', 'd');
assertEquals("dbcbccba", sb.toString());
sb.replaceFirst('b', 'e');
assertEquals("decbccba", sb.toString());
sb.replaceFirst('c', 'f');
assertEquals("defbccba", sb.toString());
sb.replaceFirst('d', 'd');
assertEquals("defbccba", sb.toString());
}
//-----------------------------------------------------------------------
public void testReplaceAll_String_String() {
StrBuilder sb = new StrBuilder("abcbccba");
sb.replaceAll((String) null, null);
assertEquals("abcbccba", sb.toString());
sb.replaceAll((String) null, "anything");
assertEquals("abcbccba", sb.toString());
sb.replaceAll("", null);
assertEquals("abcbccba", sb.toString());
sb.replaceAll("", "anything");
assertEquals("abcbccba", sb.toString());
sb.replaceAll("x", "y");
assertEquals("abcbccba", sb.toString());
sb.replaceAll("a", "d");
assertEquals("dbcbccbd", sb.toString());
sb.replaceAll("d", null);
assertEquals("bcbccb", sb.toString());
sb.replaceAll("cb", "-");
assertEquals("b-c-", sb.toString());
sb = new StrBuilder("abcba");
sb.replaceAll("b", "xbx");
assertEquals("axbxcxbxa", sb.toString());
sb = new StrBuilder("bb");
sb.replaceAll("b", "xbx");
assertEquals("xbxxbx", sb.toString());
}
public void testReplaceFirst_String_String() {
StrBuilder sb = new StrBuilder("abcbccba");
sb.replaceFirst((String) null, null);
assertEquals("abcbccba", sb.toString());
sb.replaceFirst((String) null, "anything");
assertEquals("abcbccba", sb.toString());
sb.replaceFirst("", null);
assertEquals("abcbccba", sb.toString());
sb.replaceFirst("", "anything");
assertEquals("abcbccba", sb.toString());
sb.replaceFirst("x", "y");
assertEquals("abcbccba", sb.toString());
sb.replaceFirst("a", "d");
assertEquals("dbcbccba", sb.toString());
sb.replaceFirst("d", null);
assertEquals("bcbccba", sb.toString());
sb.replaceFirst("cb", "-");
assertEquals("b-ccba", sb.toString());
sb = new StrBuilder("abcba");
sb.replaceFirst("b", "xbx");
assertEquals("axbxcba", sb.toString());
sb = new StrBuilder("bb");
sb.replaceFirst("b", "xbx");
assertEquals("xbxb", sb.toString());
}
//-----------------------------------------------------------------------
public void testReplaceAll_StrMatcher_String() {
StrBuilder sb = new StrBuilder("abcbccba");
sb.replaceAll((StrMatcher) null, null);
assertEquals("abcbccba", sb.toString());
sb.replaceAll((StrMatcher) null, "anything");
assertEquals("abcbccba", sb.toString());
sb.replaceAll(StrMatcher.noneMatcher(), null);
assertEquals("abcbccba", sb.toString());
sb.replaceAll(StrMatcher.noneMatcher(), "anything");
assertEquals("abcbccba", sb.toString());
sb.replaceAll(StrMatcher.charMatcher('x'), "y");
assertEquals("abcbccba", sb.toString());
sb.replaceAll(StrMatcher.charMatcher('a'), "d");
assertEquals("dbcbccbd", sb.toString());
sb.replaceAll(StrMatcher.charMatcher('d'), null);
assertEquals("bcbccb", sb.toString());
sb.replaceAll(StrMatcher.stringMatcher("cb"), "-");
assertEquals("b-c-", sb.toString());
sb = new StrBuilder("abcba");
sb.replaceAll(StrMatcher.charMatcher('b'), "xbx");
assertEquals("axbxcxbxa", sb.toString());
sb = new StrBuilder("bb");
sb.replaceAll(StrMatcher.charMatcher('b'), "xbx");
assertEquals("xbxxbx", sb.toString());
sb = new StrBuilder("A1-A2A3-A4");
sb.replaceAll(A_NUMBER_MATCHER, "***");
assertEquals("***-******-***", sb.toString());
}
public void testReplaceFirst_StrMatcher_String() {
StrBuilder sb = new StrBuilder("abcbccba");
sb.replaceFirst((StrMatcher) null, null);
assertEquals("abcbccba", sb.toString());
sb.replaceFirst((StrMatcher) null, "anything");
assertEquals("abcbccba", sb.toString());
sb.replaceFirst(StrMatcher.noneMatcher(), null);
assertEquals("abcbccba", sb.toString());
sb.replaceFirst(StrMatcher.noneMatcher(), "anything");
assertEquals("abcbccba", sb.toString());
sb.replaceFirst(StrMatcher.charMatcher('x'), "y");
assertEquals("abcbccba", sb.toString());
sb.replaceFirst(StrMatcher.charMatcher('a'), "d");
assertEquals("dbcbccba", sb.toString());
sb.replaceFirst(StrMatcher.charMatcher('d'), null);
assertEquals("bcbccba", sb.toString());
sb.replaceFirst(StrMatcher.stringMatcher("cb"), "-");
assertEquals("b-ccba", sb.toString());
sb = new StrBuilder("abcba");
sb.replaceFirst(StrMatcher.charMatcher('b'), "xbx");
assertEquals("axbxcba", sb.toString());
sb = new StrBuilder("bb");
sb.replaceFirst(StrMatcher.charMatcher('b'), "xbx");
assertEquals("xbxb", sb.toString());
sb = new StrBuilder("A1-A2A3-A4");
sb.replaceFirst(A_NUMBER_MATCHER, "***");
assertEquals("***-A2A3-A4", sb.toString());
}
//-----------------------------------------------------------------------
public void testReplace_StrMatcher_String_int_int_int_VaryMatcher() {
StrBuilder sb = new StrBuilder("abcbccba");
sb.replace((StrMatcher) null, "x", 0, sb.length(), -1);
assertEquals("abcbccba", sb.toString());
sb.replace(StrMatcher.charMatcher('a'), "x", 0, sb.length(), -1);
assertEquals("xbcbccbx", sb.toString());
sb.replace(StrMatcher.stringMatcher("cb"), "x", 0, sb.length(), -1);
assertEquals("xbxcxx", sb.toString());
sb = new StrBuilder("A1-A2A3-A4");
sb.replace(A_NUMBER_MATCHER, "***", 0, sb.length(), -1);
assertEquals("***-******-***", sb.toString());
sb = new StrBuilder();
sb.replace(A_NUMBER_MATCHER, "***", 0, sb.length(), -1);
assertEquals("", sb.toString());
}
public void testReplace_StrMatcher_String_int_int_int_VaryReplace() {
StrBuilder sb = new StrBuilder("abcbccba");
sb.replace(StrMatcher.stringMatcher("cb"), "cb", 0, sb.length(), -1);
assertEquals("abcbccba", sb.toString());
sb = new StrBuilder("abcbccba");
sb.replace(StrMatcher.stringMatcher("cb"), "-", 0, sb.length(), -1);
assertEquals("ab-c-a", sb.toString());
sb = new StrBuilder("abcbccba");
sb.replace(StrMatcher.stringMatcher("cb"), "+++", 0, sb.length(), -1);
assertEquals("ab+++c+++a", sb.toString());
sb = new StrBuilder("abcbccba");
sb.replace(StrMatcher.stringMatcher("cb"), "", 0, sb.length(), -1);
assertEquals("abca", sb.toString());
sb = new StrBuilder("abcbccba");
sb.replace(StrMatcher.stringMatcher("cb"), null, 0, sb.length(), -1);
assertEquals("abca", sb.toString());
}
public void testReplace_StrMatcher_String_int_int_int_VaryStartIndex() {
StrBuilder sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, sb.length(), -1);
assertEquals("-x--y-", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 1, sb.length(), -1);
assertEquals("aax--y-", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 2, sb.length(), -1);
assertEquals("aax--y-", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 3, sb.length(), -1);
assertEquals("aax--y-", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 4, sb.length(), -1);
assertEquals("aaxa-ay-", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 5, sb.length(), -1);
assertEquals("aaxaa-y-", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 6, sb.length(), -1);
assertEquals("aaxaaaay-", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 7, sb.length(), -1);
assertEquals("aaxaaaay-", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 8, sb.length(), -1);
assertEquals("aaxaaaay-", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 9, sb.length(), -1);
assertEquals("aaxaaaayaa", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 10, sb.length(), -1);
assertEquals("aaxaaaayaa", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
try {
sb.replace(StrMatcher.stringMatcher("aa"), "-", 11, sb.length(), -1);
fail();
} catch (IndexOutOfBoundsException ex) {}
assertEquals("aaxaaaayaa", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
try {
sb.replace(StrMatcher.stringMatcher("aa"), "-", -1, sb.length(), -1);
fail();
} catch (IndexOutOfBoundsException ex) {}
assertEquals("aaxaaaayaa", sb.toString());
}
public void testReplace_StrMatcher_String_int_int_int_VaryEndIndex() {
StrBuilder sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 0, -1);
assertEquals("aaxaaaayaa", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 2, -1);
assertEquals("-xaaaayaa", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 3, -1);
assertEquals("-xaaaayaa", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 4, -1);
assertEquals("-xaaaayaa", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 5, -1);
assertEquals("-x-aayaa", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 6, -1);
assertEquals("-x-aayaa", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 7, -1);
assertEquals("-x--yaa", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 8, -1);
assertEquals("-x--yaa", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 9, -1);
assertEquals("-x--yaa", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 10, -1);
assertEquals("-x--y-", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 1000, -1);
assertEquals("-x--y-", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
try {
sb.replace(StrMatcher.stringMatcher("aa"), "-", 2, 1, -1);
fail();
} catch (IndexOutOfBoundsException ex) {}
assertEquals("aaxaaaayaa", sb.toString());
}
public void testReplace_StrMatcher_String_int_int_int_VaryCount() {
StrBuilder sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 10, -1);
assertEquals("-x--y-", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 10, 0);
assertEquals("aaxaaaayaa", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 10, 1);
assertEquals("-xaaaayaa", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 10, 2);
assertEquals("-x-aayaa", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 10, 3);
assertEquals("-x--yaa", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 10, 4);
assertEquals("-x--y-", sb.toString());
sb = new StrBuilder("aaxaaaayaa");
sb.replace(StrMatcher.stringMatcher("aa"), "-", 0, 10, 5);
assertEquals("-x--y-", sb.toString());
}
//-----------------------------------------------------------------------
public void testReverse() {
StrBuilder sb = new StrBuilder();
assertEquals("", sb.reverse().toString());
sb.clear().append(true);
assertEquals("eurt", sb.reverse().toString());
assertEquals("true", sb.reverse().toString());
}
//-----------------------------------------------------------------------
public void testTrim() {
StrBuilder sb = new StrBuilder();
assertEquals("", sb.reverse().toString());
sb.clear().append(" \u0000 ");
assertEquals("", sb.trim().toString());
sb.clear().append(" \u0000 a b c");
assertEquals("a b c", sb.trim().toString());
sb.clear().append("a b c \u0000 ");
assertEquals("a b c", sb.trim().toString());
sb.clear().append(" \u0000 a b c \u0000 ");
assertEquals("a b c", sb.trim().toString());
sb.clear().append("a b c");
assertEquals("a b c", sb.trim().toString());
}
//-----------------------------------------------------------------------
public void testStartsWith() {
StrBuilder sb = new StrBuilder();
assertFalse(sb.startsWith("a"));
assertFalse(sb.startsWith(null));
assertTrue(sb.startsWith(""));
sb.append("abc");
assertTrue(sb.startsWith("a"));
assertTrue(sb.startsWith("ab"));
assertTrue(sb.startsWith("abc"));
assertFalse(sb.startsWith("cba"));
}
public void testEndsWith() {
StrBuilder sb = new StrBuilder();
assertFalse(sb.endsWith("a"));
assertFalse(sb.endsWith("c"));
assertTrue(sb.endsWith(""));
assertFalse(sb.endsWith(null));
sb.append("abc");
assertTrue(sb.endsWith("c"));
assertTrue(sb.endsWith("bc"));
assertTrue(sb.endsWith("abc"));
assertFalse(sb.endsWith("cba"));
assertFalse(sb.endsWith("abcd"));
assertFalse(sb.endsWith(" abc"));
assertFalse(sb.endsWith("abc "));
}
//-----------------------------------------------------------------------
public void testSubstringInt() {
StrBuilder sb = new StrBuilder ("hello goodbye");
assertEquals ("goodbye", sb.substring(6));
assertEquals ("hello goodbye".substring(6), sb.substring(6));
assertEquals ("hello goodbye", sb.substring(0));
assertEquals ("hello goodbye".substring(0), sb.substring(0));
try {
sb.substring(-1);
fail ();
} catch (IndexOutOfBoundsException e) {}
try {
sb.substring(15);
fail ();
} catch (IndexOutOfBoundsException e) {}
}
public void testSubstringIntInt() {
StrBuilder sb = new StrBuilder ("hello goodbye");
assertEquals ("hello", sb.substring(0, 5));
assertEquals ("hello goodbye".substring(0, 6), sb.substring(0, 6));
assertEquals ("goodbye", sb.substring(6, 13));
assertEquals ("hello goodbye".substring(6,13), sb.substring(6, 13));
assertEquals ("goodbye", sb.substring(6, 20));
try {
sb.substring(-1, 5);
fail();
} catch (IndexOutOfBoundsException e) {}
try {
sb.substring(15, 20);
fail();
} catch (IndexOutOfBoundsException e) {}
}
// -----------------------------------------------------------------------
public void testMidString() {
StrBuilder sb = new StrBuilder("hello goodbye hello");
assertEquals("goodbye", sb.midString(6, 7));
assertEquals("hello", sb.midString(0, 5));
assertEquals("hello", sb.midString(-5, 5));
assertEquals("", sb.midString(0, -1));
assertEquals("", sb.midString(20, 2));
assertEquals("hello", sb.midString(14, 22));
}
public void testRightString() {
StrBuilder sb = new StrBuilder("left right");
assertEquals("right", sb.rightString(5));
assertEquals("", sb.rightString(0));
assertEquals("", sb.rightString(-5));
assertEquals("left right", sb.rightString(15));
}
public void testLeftString() {
StrBuilder sb = new StrBuilder("left right");
assertEquals("left", sb.leftString(4));
assertEquals("", sb.leftString(0));
assertEquals("", sb.leftString(-5));
assertEquals("left right", sb.leftString(15));
}
// -----------------------------------------------------------------------
public void testContains_char() {
StrBuilder sb = new StrBuilder("abcdefghijklmnopqrstuvwxyz");
assertEquals(true, sb.contains('a'));
assertEquals(true, sb.contains('o'));
assertEquals(true, sb.contains('z'));
assertEquals(false, sb.contains('1'));
}
public void testContains_String() {
StrBuilder sb = new StrBuilder("abcdefghijklmnopqrstuvwxyz");
assertEquals(true, sb.contains("a"));
assertEquals(true, sb.contains("pq"));
assertEquals(true, sb.contains("z"));
assertEquals(false, sb.contains("zyx"));
assertEquals(false, sb.contains((String) null));
}
public void testContains_StrMatcher() {
StrBuilder sb = new StrBuilder("abcdefghijklmnopqrstuvwxyz");
assertEquals(true, sb.contains(StrMatcher.charMatcher('a')));
assertEquals(true, sb.contains(StrMatcher.stringMatcher("pq")));
assertEquals(true, sb.contains(StrMatcher.charMatcher('z')));
assertEquals(false, sb.contains(StrMatcher.stringMatcher("zy")));
assertEquals(false, sb.contains((StrMatcher) null));
sb = new StrBuilder();
assertEquals(false, sb.contains(A_NUMBER_MATCHER));
sb.append("B A1 C");
assertEquals(true, sb.contains(A_NUMBER_MATCHER));
}
// -----------------------------------------------------------------------
public void testIndexOf_char() {
StrBuilder sb = new StrBuilder("abab");
assertEquals(0, sb.indexOf('a'));
// should work like String#indexOf
assertEquals("abab".indexOf('a'), sb.indexOf('a'));
assertEquals(1, sb.indexOf('b'));
assertEquals("abab".indexOf('b'), sb.indexOf('b'));
assertEquals(-1, sb.indexOf('z'));
}
public void testIndexOf_char_int() {
StrBuilder sb = new StrBuilder("abab");
assertEquals(0, sb.indexOf('a', -1));
assertEquals(0, sb.indexOf('a', 0));
assertEquals(2, sb.indexOf('a', 1));
assertEquals(-1, sb.indexOf('a', 4));
assertEquals(-1, sb.indexOf('a', 5));
// should work like String#indexOf
assertEquals("abab".indexOf('a', 1), sb.indexOf('a', 1));
assertEquals(3, sb.indexOf('b', 2));
assertEquals("abab".indexOf('b', 2), sb.indexOf('b', 2));
assertEquals(-1, sb.indexOf('z', 2));
sb = new StrBuilder("xyzabc");
assertEquals(2, sb.indexOf('z', 0));
assertEquals(-1, sb.indexOf('z', 3));
}
public void testLastIndexOf_char() {
StrBuilder sb = new StrBuilder("abab");
assertEquals (2, sb.lastIndexOf('a'));
//should work like String#lastIndexOf
assertEquals ("abab".lastIndexOf('a'), sb.lastIndexOf('a'));
assertEquals(3, sb.lastIndexOf('b'));
assertEquals ("abab".lastIndexOf('b'), sb.lastIndexOf('b'));
assertEquals (-1, sb.lastIndexOf('z'));
}
public void testLastIndexOf_char_int() {
StrBuilder sb = new StrBuilder("abab");
assertEquals(-1, sb.lastIndexOf('a', -1));
assertEquals(0, sb.lastIndexOf('a', 0));
assertEquals(0, sb.lastIndexOf('a', 1));
// should work like String#lastIndexOf
assertEquals("abab".lastIndexOf('a', 1), sb.lastIndexOf('a', 1));
assertEquals(1, sb.lastIndexOf('b', 2));
assertEquals("abab".lastIndexOf('b', 2), sb.lastIndexOf('b', 2));
assertEquals(-1, sb.lastIndexOf('z', 2));
sb = new StrBuilder("xyzabc");
assertEquals(2, sb.lastIndexOf('z', sb.length()));
assertEquals(-1, sb.lastIndexOf('z', 1));
}
// -----------------------------------------------------------------------
public void testIndexOf_String() {
StrBuilder sb = new StrBuilder("abab");
assertEquals(0, sb.indexOf("a"));
//should work like String#indexOf
assertEquals("abab".indexOf("a"), sb.indexOf("a"));
assertEquals(0, sb.indexOf("ab"));
//should work like String#indexOf
assertEquals("abab".indexOf("ab"), sb.indexOf("ab"));
assertEquals(1, sb.indexOf("b"));
assertEquals("abab".indexOf("b"), sb.indexOf("b"));
assertEquals(1, sb.indexOf("ba"));
assertEquals("abab".indexOf("ba"), sb.indexOf("ba"));
assertEquals(-1, sb.indexOf("z"));
assertEquals(-1, sb.indexOf((String) null));
}
public void testIndexOf_String_int() {
StrBuilder sb = new StrBuilder("abab");
assertEquals(0, sb.indexOf("a", -1));
assertEquals(0, sb.indexOf("a", 0));
assertEquals(2, sb.indexOf("a", 1));
assertEquals(2, sb.indexOf("a", 2));
assertEquals(-1, sb.indexOf("a", 3));
assertEquals(-1, sb.indexOf("a", 4));
assertEquals(-1, sb.indexOf("a", 5));
assertEquals(-1, sb.indexOf("abcdef", 0));
assertEquals(0, sb.indexOf("", 0));
assertEquals(1, sb.indexOf("", 1));
//should work like String#indexOf
assertEquals ("abab".indexOf("a", 1), sb.indexOf("a", 1));
assertEquals(2, sb.indexOf("ab", 1));
//should work like String#indexOf
assertEquals("abab".indexOf("ab", 1), sb.indexOf("ab", 1));
assertEquals(3, sb.indexOf("b", 2));
assertEquals("abab".indexOf("b", 2), sb.indexOf("b", 2));
assertEquals(1, sb.indexOf("ba", 1));
assertEquals("abab".indexOf("ba", 2), sb.indexOf("ba", 2));
assertEquals(-1, sb.indexOf("z", 2));
sb = new StrBuilder("xyzabc");
assertEquals(2, sb.indexOf("za", 0));
assertEquals(-1, sb.indexOf("za", 3));
assertEquals(-1, sb.indexOf((String) null, 2));
}
public void testLastIndexOf_String() {
StrBuilder sb = new StrBuilder("abab");
assertEquals(2, sb.lastIndexOf("a"));
//should work like String#lastIndexOf
assertEquals("abab".lastIndexOf("a"), sb.lastIndexOf("a"));
assertEquals(2, sb.lastIndexOf("ab"));
//should work like String#lastIndexOf
assertEquals("abab".lastIndexOf("ab"), sb.lastIndexOf("ab"));
assertEquals(3, sb.lastIndexOf("b"));
assertEquals("abab".lastIndexOf("b"), sb.lastIndexOf("b"));
assertEquals(1, sb.lastIndexOf("ba"));
assertEquals("abab".lastIndexOf("ba"), sb.lastIndexOf("ba"));
assertEquals(-1, sb.lastIndexOf("z"));
assertEquals(-1, sb.lastIndexOf((String) null));
}
public void testLastIndexOf_String_int() {
StrBuilder sb = new StrBuilder("abab");
assertEquals(-1, sb.lastIndexOf("a", -1));
assertEquals(0, sb.lastIndexOf("a", 0));
assertEquals(0, sb.lastIndexOf("a", 1));
assertEquals(2, sb.lastIndexOf("a", 2));
assertEquals(2, sb.lastIndexOf("a", 3));
assertEquals(2, sb.lastIndexOf("a", 4));
assertEquals(2, sb.lastIndexOf("a", 5));
assertEquals(-1, sb.lastIndexOf("abcdef", 3));
assertEquals("abab".lastIndexOf("", 3), sb.lastIndexOf("", 3));
assertEquals("abab".lastIndexOf("", 1), sb.lastIndexOf("", 1));
//should work like String#lastIndexOf
assertEquals("abab".lastIndexOf("a", 1), sb.lastIndexOf("a", 1));
assertEquals(0, sb.lastIndexOf("ab", 1));
//should work like String#lastIndexOf
assertEquals("abab".lastIndexOf("ab", 1), sb.lastIndexOf("ab", 1));
assertEquals(1, sb.lastIndexOf("b", 2));
assertEquals("abab".lastIndexOf("b", 2), sb.lastIndexOf("b", 2));
assertEquals(1, sb.lastIndexOf("ba", 2));
assertEquals("abab".lastIndexOf("ba", 2), sb.lastIndexOf("ba", 2));
assertEquals(-1, sb.lastIndexOf("z", 2));
sb = new StrBuilder("xyzabc");
assertEquals(2, sb.lastIndexOf("za", sb.length()));
assertEquals(-1, sb.lastIndexOf("za", 1));
assertEquals(-1, sb.lastIndexOf((String) null, 2));
}
// -----------------------------------------------------------------------
public void testIndexOf_StrMatcher() {
StrBuilder sb = new StrBuilder();
assertEquals(-1, sb.indexOf((StrMatcher) null));
assertEquals(-1, sb.indexOf(StrMatcher.charMatcher('a')));
sb.append("ab bd");
assertEquals(0, sb.indexOf(StrMatcher.charMatcher('a')));
assertEquals(1, sb.indexOf(StrMatcher.charMatcher('b')));
assertEquals(2, sb.indexOf(StrMatcher.spaceMatcher()));
assertEquals(4, sb.indexOf(StrMatcher.charMatcher('d')));
assertEquals(-1, sb.indexOf(StrMatcher.noneMatcher()));
assertEquals(-1, sb.indexOf((StrMatcher) null));
sb.append(" A1 junction");
assertEquals(6, sb.indexOf(A_NUMBER_MATCHER));
}
public void testIndexOf_StrMatcher_int() {
StrBuilder sb = new StrBuilder();
assertEquals(-1, sb.indexOf((StrMatcher) null, 2));
assertEquals(-1, sb.indexOf(StrMatcher.charMatcher('a'), 2));
assertEquals(-1, sb.indexOf(StrMatcher.charMatcher('a'), 0));
sb.append("ab bd");
assertEquals(0, sb.indexOf(StrMatcher.charMatcher('a'), -2));
assertEquals(0, sb.indexOf(StrMatcher.charMatcher('a'), 0));
assertEquals(-1, sb.indexOf(StrMatcher.charMatcher('a'), 2));
assertEquals(-1, sb.indexOf(StrMatcher.charMatcher('a'), 20));
assertEquals(1, sb.indexOf(StrMatcher.charMatcher('b'), -1));
assertEquals(1, sb.indexOf(StrMatcher.charMatcher('b'), 0));
assertEquals(1, sb.indexOf(StrMatcher.charMatcher('b'), 1));
assertEquals(3, sb.indexOf(StrMatcher.charMatcher('b'), 2));
assertEquals(3, sb.indexOf(StrMatcher.charMatcher('b'), 3));
assertEquals(-1, sb.indexOf(StrMatcher.charMatcher('b'), 4));
assertEquals(-1, sb.indexOf(StrMatcher.charMatcher('b'), 5));
assertEquals(-1, sb.indexOf(StrMatcher.charMatcher('b'), 6));
assertEquals(2, sb.indexOf(StrMatcher.spaceMatcher(), -2));
assertEquals(2, sb.indexOf(StrMatcher.spaceMatcher(), 0));
assertEquals(2, sb.indexOf(StrMatcher.spaceMatcher(), 2));
assertEquals(-1, sb.indexOf(StrMatcher.spaceMatcher(), 4));
assertEquals(-1, sb.indexOf(StrMatcher.spaceMatcher(), 20));
assertEquals(-1, sb.indexOf(StrMatcher.noneMatcher(), 0));
assertEquals(-1, sb.indexOf((StrMatcher) null, 0));
sb.append(" A1 junction with A2");
assertEquals(6, sb.indexOf(A_NUMBER_MATCHER, 5));
assertEquals(6, sb.indexOf(A_NUMBER_MATCHER, 6));
assertEquals(23, sb.indexOf(A_NUMBER_MATCHER, 7));
assertEquals(23, sb.indexOf(A_NUMBER_MATCHER, 22));
assertEquals(23, sb.indexOf(A_NUMBER_MATCHER, 23));
assertEquals(-1, sb.indexOf(A_NUMBER_MATCHER, 24));
}
public void testLastIndexOf_StrMatcher() {
StrBuilder sb = new StrBuilder();
assertEquals(-1, sb.lastIndexOf((StrMatcher) null));
assertEquals(-1, sb.lastIndexOf(StrMatcher.charMatcher('a')));
sb.append("ab bd");
assertEquals(0, sb.lastIndexOf(StrMatcher.charMatcher('a')));
assertEquals(3, sb.lastIndexOf(StrMatcher.charMatcher('b')));
assertEquals(2, sb.lastIndexOf(StrMatcher.spaceMatcher()));
assertEquals(4, sb.lastIndexOf(StrMatcher.charMatcher('d')));
assertEquals(-1, sb.lastIndexOf(StrMatcher.noneMatcher()));
assertEquals(-1, sb.lastIndexOf((StrMatcher) null));
sb.append(" A1 junction");
assertEquals(6, sb.lastIndexOf(A_NUMBER_MATCHER));
}
public void testLastIndexOf_StrMatcher_int() {
StrBuilder sb = new StrBuilder();
assertEquals(-1, sb.lastIndexOf((StrMatcher) null, 2));
assertEquals(-1, sb.lastIndexOf(StrMatcher.charMatcher('a'), 2));
assertEquals(-1, sb.lastIndexOf(StrMatcher.charMatcher('a'), 0));
assertEquals(-1, sb.lastIndexOf(StrMatcher.charMatcher('a'), -1));
sb.append("ab bd");
assertEquals(-1, sb.lastIndexOf(StrMatcher.charMatcher('a'), -2));
assertEquals(0, sb.lastIndexOf(StrMatcher.charMatcher('a'), 0));
assertEquals(0, sb.lastIndexOf(StrMatcher.charMatcher('a'), 2));
assertEquals(0, sb.lastIndexOf(StrMatcher.charMatcher('a'), 20));
assertEquals(-1, sb.lastIndexOf(StrMatcher.charMatcher('b'), -1));
assertEquals(-1, sb.lastIndexOf(StrMatcher.charMatcher('b'), 0));
assertEquals(1, sb.lastIndexOf(StrMatcher.charMatcher('b'), 1));
assertEquals(1, sb.lastIndexOf(StrMatcher.charMatcher('b'), 2));
assertEquals(3, sb.lastIndexOf(StrMatcher.charMatcher('b'), 3));
assertEquals(3, sb.lastIndexOf(StrMatcher.charMatcher('b'), 4));
assertEquals(3, sb.lastIndexOf(StrMatcher.charMatcher('b'), 5));
assertEquals(3, sb.lastIndexOf(StrMatcher.charMatcher('b'), 6));
assertEquals(-1, sb.lastIndexOf(StrMatcher.spaceMatcher(), -2));
assertEquals(-1, sb.lastIndexOf(StrMatcher.spaceMatcher(), 0));
assertEquals(2, sb.lastIndexOf(StrMatcher.spaceMatcher(), 2));
assertEquals(2, sb.lastIndexOf(StrMatcher.spaceMatcher(), 4));
assertEquals(2, sb.lastIndexOf(StrMatcher.spaceMatcher(), 20));
assertEquals(-1, sb.lastIndexOf(StrMatcher.noneMatcher(), 0));
assertEquals(-1, sb.lastIndexOf((StrMatcher) null, 0));
sb.append(" A1 junction with A2");
assertEquals(-1, sb.lastIndexOf(A_NUMBER_MATCHER, 5));
assertEquals(-1, sb.lastIndexOf(A_NUMBER_MATCHER, 6)); // A matches, 1 is outside bounds
assertEquals(6, sb.lastIndexOf(A_NUMBER_MATCHER, 7));
assertEquals(6, sb.lastIndexOf(A_NUMBER_MATCHER, 22));
assertEquals(6, sb.lastIndexOf(A_NUMBER_MATCHER, 23)); // A matches, 2 is outside bounds
assertEquals(23, sb.lastIndexOf(A_NUMBER_MATCHER, 24));
}
static final StrMatcher A_NUMBER_MATCHER = new StrMatcher() {
public int isMatch(char[] buffer, int pos, int bufferStart, int bufferEnd) {
if (buffer[pos] == 'A') {
pos++;
if (pos < bufferEnd && buffer[pos] >= '0' && buffer[pos] <= '9') {
return 2;
}
}
return 0;
}
};
//-----------------------------------------------------------------------
public void testAsTokenizer() throws Exception {
// from Javadoc
StrBuilder b = new StrBuilder();
b.append("a b ");
StrTokenizer t = b.asTokenizer();
String[] tokens1 = t.getTokenArray();
assertEquals(2, tokens1.length);
assertEquals("a", tokens1[0]);
assertEquals("b", tokens1[1]);
assertEquals(2, t.size());
b.append("c d ");
String[] tokens2 = t.getTokenArray();
assertEquals(2, tokens2.length);
assertEquals("a", tokens2[0]);
assertEquals("b", tokens2[1]);
assertEquals(2, t.size());
assertEquals("a", t.next());
assertEquals("b", t.next());
t.reset();
String[] tokens3 = t.getTokenArray();
assertEquals(4, tokens3.length);
assertEquals("a", tokens3[0]);
assertEquals("b", tokens3[1]);
assertEquals("c", tokens3[2]);
assertEquals("d", tokens3[3]);
assertEquals(4, t.size());
assertEquals("a", t.next());
assertEquals("b", t.next());
assertEquals("c", t.next());
assertEquals("d", t.next());
assertEquals("a b c d ", t.getContent());
}
// -----------------------------------------------------------------------
public void testAsReader() throws Exception {
StrBuilder sb = new StrBuilder("some text");
Reader reader = sb.asReader();
assertEquals(true, reader.ready());
char[] buf = new char[40];
assertEquals(9, reader.read(buf));
assertEquals("some text", new String(buf, 0, 9));
assertEquals(-1, reader.read());
assertEquals(false, reader.ready());
assertEquals(0, reader.skip(2));
assertEquals(0, reader.skip(-1));
assertEquals(true, reader.markSupported());
reader = sb.asReader();
assertEquals('s', reader.read());
reader.mark(-1);
char[] array = new char[3];
assertEquals(3, reader.read(array, 0, 3));
assertEquals('o', array[0]);
assertEquals('m', array[1]);
assertEquals('e', array[2]);
reader.reset();
assertEquals(1, reader.read(array, 1, 1));
assertEquals('o', array[0]);
assertEquals('o', array[1]);
assertEquals('e', array[2]);
assertEquals(2, reader.skip(2));
assertEquals(' ', reader.read());
assertEquals(true, reader.ready());
reader.close();
assertEquals(true, reader.ready());
reader = sb.asReader();
array = new char[3];
try {
reader.read(array, -1, 0);
fail();
} catch (IndexOutOfBoundsException ex) {}
try {
reader.read(array, 0, -1);
fail();
} catch (IndexOutOfBoundsException ex) {}
try {
reader.read(array, 100, 1);
fail();
} catch (IndexOutOfBoundsException ex) {}
try {
reader.read(array, 0, 100);
fail();
} catch (IndexOutOfBoundsException ex) {}
try {
reader.read(array, Integer.MAX_VALUE, Integer.MAX_VALUE);
fail();
} catch (IndexOutOfBoundsException ex) {}
assertEquals(0, reader.read(array, 0, 0));
assertEquals(0, array[0]);
assertEquals(0, array[1]);
assertEquals(0, array[2]);
reader.skip(9);
assertEquals(-1, reader.read(array, 0, 1));
reader.reset();
array = new char[30];
assertEquals(9, reader.read(array, 0, 30));
}
//-----------------------------------------------------------------------
public void testAsWriter() throws Exception {
StrBuilder sb = new StrBuilder("base");
Writer writer = sb.asWriter();
writer.write('l');
assertEquals("basel", sb.toString());
writer.write(new char[] {'i', 'n'});
assertEquals("baselin", sb.toString());
writer.write(new char[] {'n', 'e', 'r'}, 1, 2);
assertEquals("baseliner", sb.toString());
writer.write(" rout");
assertEquals("baseliner rout", sb.toString());
writer.write("ping that server", 1, 3);
assertEquals("baseliner routing", sb.toString());
writer.flush(); // no effect
assertEquals("baseliner routing", sb.toString());
writer.close(); // no effect
assertEquals("baseliner routing", sb.toString());
writer.write(" hi"); // works after close
assertEquals("baseliner routing hi", sb.toString());
sb.setLength(4); // mix and match
writer.write('d');
assertEquals("based", sb.toString());
}
//-----------------------------------------------------------------------
public void testEqualsIgnoreCase() {
StrBuilder sb1 = new StrBuilder();
StrBuilder sb2 = new StrBuilder();
assertEquals(true, sb1.equalsIgnoreCase(sb1));
assertEquals(true, sb1.equalsIgnoreCase(sb2));
assertEquals(true, sb2.equalsIgnoreCase(sb2));
sb1.append("abc");
assertEquals(false, sb1.equalsIgnoreCase(sb2));
sb2.append("ABC");
assertEquals(true, sb1.equalsIgnoreCase(sb2));
sb2.clear().append("abc");
assertEquals(true, sb1.equalsIgnoreCase(sb2));
assertEquals(true, sb1.equalsIgnoreCase(sb1));
assertEquals(true, sb2.equalsIgnoreCase(sb2));
sb2.clear().append("aBc");
assertEquals(true, sb1.equalsIgnoreCase(sb2));
}
//-----------------------------------------------------------------------
public void testEquals() {
StrBuilder sb1 = new StrBuilder();
StrBuilder sb2 = new StrBuilder();
assertEquals(true, sb1.equals(sb2));
assertEquals(true, sb1.equals(sb1));
assertEquals(true, sb2.equals(sb2));
assertEquals(true, sb1.equals((Object) sb2));
sb1.append("abc");
assertEquals(false, sb1.equals(sb2));
assertEquals(false, sb1.equals((Object) sb2));
sb2.append("ABC");
assertEquals(false, sb1.equals(sb2));
assertEquals(false, sb1.equals((Object) sb2));
sb2.clear().append("abc");
assertEquals(true, sb1.equals(sb2));
assertEquals(true, sb1.equals((Object) sb2));
assertEquals(false, sb1.equals(new Integer(1)));
assertEquals(false, sb1.equals("abc"));
}
//-----------------------------------------------------------------------
public void testHashCode() {
StrBuilder sb = new StrBuilder();
int hc1a = sb.hashCode();
int hc1b = sb.hashCode();
assertEquals(0, hc1a);
assertEquals(hc1a, hc1b);
sb.append("abc");
int hc2a = sb.hashCode();
int hc2b = sb.hashCode();
assertEquals(true, hc2a != 0);
assertEquals(hc2a, hc2b);
}
//-----------------------------------------------------------------------
public void testToString() {
StrBuilder sb = new StrBuilder("abc");
assertEquals("abc", sb.toString());
}
//-----------------------------------------------------------------------
public void testToStringBuffer() {
StrBuilder sb = new StrBuilder();
assertEquals(new StringBuffer().toString(), sb.toStringBuffer().toString());
sb.append("junit");
assertEquals(new StringBuffer("junit").toString(), sb.toStringBuffer().toString());
}
//-----------------------------------------------------------------------
public void testLang294() {
StrBuilder sb = new StrBuilder("\n%BLAH%\nDo more stuff\neven more stuff\n%BLAH%\n");
sb.deleteAll("\n%BLAH%");
assertEquals("\nDo more stuff\neven more stuff\n", sb.toString());
}
public void testIndexOfLang294() {
StrBuilder sb = new StrBuilder("onetwothree");
sb.deleteFirst("three");
assertEquals(-1, sb.indexOf("three"));
}
} |