input
stringlengths 50
13.9k
| output_program
stringlengths 5
655k
| output_answer
stringlengths 5
655k
| split
stringclasses 1
value | dataset
stringclasses 1
value |
---|---|---|---|---|
When Serezha was three years old, he was given a set of cards with letters for his birthday. They were arranged into words in the way which formed the boy's mother favorite number in binary notation. Serezha started playing with them immediately and shuffled them because he wasn't yet able to read. His father decided to rearrange them. Help him restore the original number, on condition that it was the maximum possible one.
-----Input-----
The first line contains a single integer $n$ ($1 \leqslant n \leqslant 10^5$) — the length of the string. The second line contains a string consisting of English lowercase letters: 'z', 'e', 'r', 'o' and 'n'.
It is guaranteed that it is possible to rearrange the letters in such a way that they form a sequence of words, each being either "zero" which corresponds to the digit $0$ or "one" which corresponds to the digit $1$.
-----Output-----
Print the maximum possible number in binary notation. Print binary digits separated by a space. The leading zeroes are allowed.
-----Examples-----
Input
4
ezor
Output
0
Input
10
nznooeeoer
Output
1 1 0
-----Note-----
In the first example, the correct initial ordering is "zero".
In the second example, the correct initial ordering is "oneonezero". | def main():
import sys
input = sys.stdin.readline
n = int(input())
arr = input()
one = arr.count('n')
zero = arr.count('z')
ans = [1] * one + [0] * zero
print(*ans)
return 0
main()
| def main():
import sys
input = sys.stdin.readline
n = int(input())
arr = input()
one = arr.count('n')
zero = arr.count('z')
ans = [1] * one + [0] * zero
print(*ans)
return 0
main()
| train | APPS_structured |
Unicode Transformation Format – 8-bit
As the name suggests UTF-8 was designed to encode data in a stream of bytes.
It works by splitting the bits up in multiples of eight. This is achieved by inserting headers to mark in how many bytes the bits were split. If the bits need to be split in two, the header `110` is added as prefix leaving five bits of the byte for the rest of the data. Followed by a continuation byte.
A continuation byte always start with `10` leaving six bits for data.
For a three-way split: the header `1110` is added with two continuation bytes and for four: `11110` with three continuation bytes. The number of ones at the start of the first byte denotes the number of bytes the data was split in.
# Task
Your task is to write two functions:
1. `to_utf8_binary`: which encodes a string to a bitstring using UTF-8 encoding.
2. `from_utf8_binary`: which does the reverse.
- Layout of UTF-8 byte sequences:
```
# BYTES FIRST CODE POINT LAST CODE POINT BYTE 1 BYTE 2 BYTE 3 BYTE 4
1 0 127 0xxxxxxx
2 128 2047 110xxxxx 10xxxxxx
3 2048 65535 1110xxxx 10xxxxxx 10xxxxxx
4 65536 1114111 11110xxx 10xxxxxx 10xxxxxx 10xxxxxx
```
# Examples
```
ENCODE
A -> 1000001 -> 01000001
八 -> 101000101101011 -> 1110-0101 10-000101 10-101011
DECODE
110-00010 10-100111 -> 10100111 -> §
11110-000 10-010000 10-001010 10-001100 -> 10000001010001100 -> 𐊌
```
* Spaces and hyphens just for clarity
- https://en.wikipedia.org/wiki/UTF-8#Encoding | def to_utf8_binary(string):
return ''.join((bin(i)[2:]).zfill(8) for i in string.encode('utf-8'))
def from_utf8_binary(bitstr):
byte_str = bytes(int(bitstr[i:i + 8], 2) for i in range(0, len(bitstr), 8))
return byte_str.decode('utf-8') | def to_utf8_binary(string):
return ''.join((bin(i)[2:]).zfill(8) for i in string.encode('utf-8'))
def from_utf8_binary(bitstr):
byte_str = bytes(int(bitstr[i:i + 8], 2) for i in range(0, len(bitstr), 8))
return byte_str.decode('utf-8') | train | APPS_structured |
Take a sentence (string) and reverse each word in the sentence. Do not reverse the order of the words, just the letters in each word.
If there is punctuation, it should be interpreted as a regular character; no special rules.
If there is spacing before/after the input string, leave them there.
String will not be empty.
## Examples
```
"Hi mom" => "iH mom"
" A fun little challenge! " => " A nuf elttil !egnellahc "
``` | def reverser(sentence):
newsent = sentence.split(" ")
rnsent = [i[::-1] for i in newsent]
s = " "
s = s.join(rnsent)
return s | def reverser(sentence):
newsent = sentence.split(" ")
rnsent = [i[::-1] for i in newsent]
s = " "
s = s.join(rnsent)
return s | train | APPS_structured |
# 'Magic' recursion call depth number
This Kata was designed as a Fork to the one from donaldsebleung Roboscript series with a reference to:
https://www.codewars.com/collections/roboscript
It is not more than an extension of Roboscript infinite "single-" mutual recursion handling to a "multiple-" case.
One can suppose that you have a machine that works through a specific language. It uses the script, which consists of 3 major commands:
- `F` - Move forward by 1 step in the direction that it is currently pointing.
- `L` - Turn "left" (i.e. rotate 90 degrees anticlockwise).
- `R` - Turn "right" (i.e. rotate 90 degrees clockwise).
The number n afterwards enforces the command to execute n times.
To improve its efficiency machine language is enriched by patterns that are containers to pack and unpack the script.
The basic syntax for defining a pattern is as follows:
`pnq`
Where:
- `p` is a "keyword" that declares the beginning of a pattern definition
- `n` is a non-negative integer, which acts as a unique identifier for the pattern (pay attention, it may contain several digits).
- `` is a valid RoboScript code (without the angled brackets)
- `q` is a "keyword" that marks the end of a pattern definition
For example, if you want to define `F2LF2` as a pattern and reuse it later:
```
p333F2LF2q
```
To invoke a pattern, a capital `P` followed by the pattern identifier `(n)` is used:
```
P333
```
It doesn't matter whether the invocation of the pattern or the pattern definition comes first. Pattern definitions should always be parsed first.
```
P333p333P11F2LF2qP333p11FR5Lq
```
# ___Infinite recursion___
As we don't want a robot to be damaged or damaging someone else by becoming uncontrolable when stuck in an infinite loop, it's good to considere this possibility in the programs and to build a compiler that can detect such potential troubles before they actually happen.
* ### Single pattern recursion infinite loop
This is the simplest case, that occurs when the pattern is invoked inside its definition:
p333P333qP333 => depth = 1: P333 -> (P333)
* ### Single mutual recursion infinite loop
Occurs when a pattern calls to unpack the mutual one, which contains a callback to the first:
p1P2qp2P1qP2 => depth = 2: P2 -> P1 -> (P2)
* ### Multiple mutual recursion infinite loop
Occurs within the combo set of mutual callbacks without termination:
p1P2qp2P3qp3P1qP3 => depth = 3: P3 -> P1 -> P2 -> (P3)
* ### No infinite recursion: terminating branch
This happens when the program can finish without encountering an infinite loop. Meaning the depth will be considered 0. Some examples below:
P4p4FLRq => depth = 0
p1P2qp2R5qP1 => depth = 0
p1P2qp2P1q => depth = 0 (no call)
# Task
Your interpreter should be able to analyse infinite recursion profiles in the input program, including multi-mutual cases.
Though, rather than to analyse only the first encountered infinite loop and get stuck in it like the robot would be, your code will have continue deeper in the calls to find the depth of any infinite recursion or terminating call. Then it should return the minimal and the maximal depths encountered, as an array `[min, max]`.
### About the exploration of the different possible branches of the program:
* Consider only patterns that are to be executed:
```
p1P1q => should return [0, 0], there is no execution
p1P2P3qp2P1qp3P1q => similarly [0, 0]
p1P1qP1 => returns [1, 1]
```
* All patterns need to be executed, strictly left to right. Meaning that you may encounter several branches:
```
p1P2P3qp2P1qp3P1qP3 => should return [2, 3]
P3 -> P1 -> P2 -> (P1) depth = 3 (max)
\-> (P3) depth = 2 (min)
```
# Input
* A valid RoboScript program, as string.
* Nested definitions of patterns, such as `p1...p2***q...q` will not be tested, even if that could be of interest as a Roboscript improvement.
* All patterns will have a unique identifier.
* Since the program is valid, you won't encounter calls to undefined pattern either.
# Output
* An array `[min, max]`, giving what are the minimal and the maximal recursion depths encountered.
### Examples
```
p1F2RF2LqP1 => should return [0, 0], no infinite recursion detected
p1F2RP1F2LqP1 => should return [1, 1], infinite recursion detection case
P2p1P2qp2P1q => should return [2, 2], single mutual infinite recursion case
p1P2qP3p2P3qp3P1q => should return [3, 3], twice mutual infinite recursion case
p1P2P1qp2P3qp3P1qP1 => should return [1, 3], mixed infinite recursion case
``` | import re
class Pattern:
def __init__(self, definition, patterns):
self._name = re.findall("p([0-9]+)", definition)[0]
self._calls = re.findall("P([0-9]+)", definition)
patterns[self._name] = self
def simulate(self, patterns, visited, depths, depth=0):
if self._name in visited:
depths.append(depth)
return
if len(self._calls) == 0:
depths.append(0)
return
visited.add(self._name)
for call in self._calls:
patterns[call].simulate(patterns, visited, depths, depth+1)
visited.remove(self._name)
def magic_call_depth_number(pattern):
patterns = {}
while True:
match = re.search("p[0-9]+[^q]*q", pattern)
if match == None:
break
(start, end) = match.span()
pattern = pattern[0:start] + pattern[end:]
Pattern(match.group(0), patterns)
depths = []
for e in re.findall("P([0-9]+)", pattern):
visited = set()
patterns[e].simulate(patterns, visited, depths)
if len(depths) == 0:
return [0, 0]
else:
return [min(depths), max(depths)] | import re
class Pattern:
def __init__(self, definition, patterns):
self._name = re.findall("p([0-9]+)", definition)[0]
self._calls = re.findall("P([0-9]+)", definition)
patterns[self._name] = self
def simulate(self, patterns, visited, depths, depth=0):
if self._name in visited:
depths.append(depth)
return
if len(self._calls) == 0:
depths.append(0)
return
visited.add(self._name)
for call in self._calls:
patterns[call].simulate(patterns, visited, depths, depth+1)
visited.remove(self._name)
def magic_call_depth_number(pattern):
patterns = {}
while True:
match = re.search("p[0-9]+[^q]*q", pattern)
if match == None:
break
(start, end) = match.span()
pattern = pattern[0:start] + pattern[end:]
Pattern(match.group(0), patterns)
depths = []
for e in re.findall("P([0-9]+)", pattern):
visited = set()
patterns[e].simulate(patterns, visited, depths)
if len(depths) == 0:
return [0, 0]
else:
return [min(depths), max(depths)] | train | APPS_structured |
Complete the method that takes a sequence of objects with two keys each: country or state, and capital. Keys may be symbols or strings.
The method should return an array of sentences declaring the state or country and its capital.
## Examples
```python
[{'state': 'Maine', 'capital': 'Augusta'}] --> ["The capital of Maine is Augusta"]
[{'country' : 'Spain', 'capital' : 'Madrid'}] --> ["The capital of Spain is Madrid"]
[{"state" : 'Maine', 'capital': 'Augusta'}, {'country': 'Spain', "capital" : "Madrid"}] --> ["The capital of Maine is Augusta", "The capital of Spain is Madrid"]
``` | def capital(capitals):
list1 = []
for i in capitals:
a = list(i.values())
print(a)
list1.append("The capital of " + a[0] + " is " + a[1])
return list1
| def capital(capitals):
list1 = []
for i in capitals:
a = list(i.values())
print(a)
list1.append("The capital of " + a[0] + " is " + a[1])
return list1
| train | APPS_structured |
Given a string s of '(' , ')' and lowercase English characters.
Your task is to remove the minimum number of parentheses ( '(' or ')', in any positions ) so that the resulting parentheses string is valid and return any valid string.
Formally, a parentheses string is valid if and only if:
It is the empty string, contains only lowercase characters, or
It can be written as AB (A concatenated with B), where A and B are valid strings, or
It can be written as (A), where A is a valid string.
Example 1:
Input: s = "lee(t(c)o)de)"
Output: "lee(t(c)o)de"
Explanation: "lee(t(co)de)" , "lee(t(c)ode)" would also be accepted.
Example 2:
Input: s = "a)b(c)d"
Output: "ab(c)d"
Example 3:
Input: s = "))(("
Output: ""
Explanation: An empty string is also valid.
Example 4:
Input: s = "(a(b(c)d)"
Output: "a(b(c)d)"
Constraints:
1 <= s.length <= 10^5
s[i] is one of '(' , ')' and lowercase English letters. | class Solution:
def minRemoveToMakeValid(self, s: str) -> str:
par_stack = []
for i, ch in enumerate(s):
if ch == '(' or ch == ')':
par_stack.append((i, ch))
while len(par_stack) >= 2 and par_stack[-1][1] == ')' and par_stack[-2][1] == '(':
par_stack.pop()
par_stack.pop()
invalid = {x[0]: True for x in par_stack}
ret = [ch for i, ch in enumerate(s) if i not in invalid]
return ''.join(ret) | class Solution:
def minRemoveToMakeValid(self, s: str) -> str:
par_stack = []
for i, ch in enumerate(s):
if ch == '(' or ch == ')':
par_stack.append((i, ch))
while len(par_stack) >= 2 and par_stack[-1][1] == ')' and par_stack[-2][1] == '(':
par_stack.pop()
par_stack.pop()
invalid = {x[0]: True for x in par_stack}
ret = [ch for i, ch in enumerate(s) if i not in invalid]
return ''.join(ret) | train | APPS_structured |
After learning a lot about space exploration, a little girl named Ana wants to change the subject.
Ana is a girl who loves palindromes (string that can be read the same backwards as forward). She has learned how to check for a given string whether it's a palindrome or not, but soon she grew tired of this problem, so she came up with a more interesting one and she needs your help to solve it:
You are given an array of strings which consist of only small letters of the alphabet. Your task is to find how many palindrome pairs are there in the array. A palindrome pair is a pair of strings such that the following condition holds: at least one permutation of the concatenation of the two strings is a palindrome. In other words, if you have two strings, let's say "aab" and "abcac", and you concatenate them into "aababcac", we have to check if there exists a permutation of this new string such that it is a palindrome (in this case there exists the permutation "aabccbaa").
Two pairs are considered different if the strings are located on different indices. The pair of strings with indices $(i,j)$ is considered the same as the pair $(j,i)$.
-----Input-----
The first line contains a positive integer $N$ ($1 \le N \le 100\,000$), representing the length of the input array.
Eacg of the next $N$ lines contains a string (consisting of lowercase English letters from 'a' to 'z') — an element of the input array.
The total number of characters in the input array will be less than $1\,000\,000$.
-----Output-----
Output one number, representing how many palindrome pairs there are in the array.
-----Examples-----
Input
3
aa
bb
cd
Output
1
Input
6
aab
abcac
dffe
ed
aa
aade
Output
6
-----Note-----
The first example: aa $+$ bb $\to$ abba.
The second example: aab $+$ abcac $=$ aababcac $\to$ aabccbaa aab $+$ aa $=$ aabaa abcac $+$ aa $=$ abcacaa $\to$ aacbcaa dffe $+$ ed $=$ dffeed $\to$ fdeedf dffe $+$ aade $=$ dffeaade $\to$ adfaafde ed $+$ aade $=$ edaade $\to$ aeddea | def main():
n = int(input())
ans = 0
d = {}
for i in range(n):
s = input()
cur = 0
for c in s:
cur ^= (1 << (ord(c) - ord('a')))
ans += d.get(cur, 0)
for j in range(26):
ans += d.get(cur ^ (1 << j), 0)
t = d.get(cur, 0) + 1
d[cur] = t
print(ans)
return
def __starting_point():
main()
__starting_point() | def main():
n = int(input())
ans = 0
d = {}
for i in range(n):
s = input()
cur = 0
for c in s:
cur ^= (1 << (ord(c) - ord('a')))
ans += d.get(cur, 0)
for j in range(26):
ans += d.get(cur ^ (1 << j), 0)
t = d.get(cur, 0) + 1
d[cur] = t
print(ans)
return
def __starting_point():
main()
__starting_point() | train | APPS_structured |
The look and say sequence is a sequence in which each number is the result of a "look and say" operation on the previous element.
Considering for example the classical version startin with `"1"`: `["1", "11", "21, "1211", "111221", ...]`. You can see that the second element describes the first as `"1(times number)1"`, the third is `"2(times number)1"` describing the second, the fourth is `"1(times number)2(and)1(times number)1"` and so on.
Your goal is to create a function which takes a starting string (not necessarily the classical `"1"`, much less a single character start) and return the nth element of the series.
## Examples
```python
look_and_say_sequence("1", 1) == "1"
look_and_say_sequence("1", 3) == "21"
look_and_say_sequence("1", 5) == "111221"
look_and_say_sequence("22", 10) == "22"
look_and_say_sequence("14", 2) == "1114"
```
Trivia: `"22"` is the only element that can keep the series constant. | import re
def look_and_say_sequence(data, n):
return data if n == 1 else look_and_say_sequence(''.join(f'{len(e.group(0))}{e.group(1)}' for e in re.finditer(r"(\d)\1*", data)), n-1)
| import re
def look_and_say_sequence(data, n):
return data if n == 1 else look_and_say_sequence(''.join(f'{len(e.group(0))}{e.group(1)}' for e in re.finditer(r"(\d)\1*", data)), n-1)
| train | APPS_structured |
Evlampiy has found one more cool application to process photos. However the application has certain limitations.
Each photo i has a contrast v_{i}. In order for the processing to be truly of high quality, the application must receive at least k photos with contrasts which differ as little as possible.
Evlampiy already knows the contrast v_{i} for each of his n photos. Now he wants to split the photos into groups, so that each group contains at least k photos. As a result, each photo must belong to exactly one group.
He considers a processing time of the j-th group to be the difference between the maximum and minimum values of v_{i} in the group. Because of multithreading the processing time of a division into groups is the maximum processing time among all groups.
Split n photos into groups in a such way that the processing time of the division is the minimum possible, i.e. that the the maximum processing time over all groups as least as possible.
-----Input-----
The first line contains two integers n and k (1 ≤ k ≤ n ≤ 3·10^5) — number of photos and minimum size of a group.
The second line contains n integers v_1, v_2, ..., v_{n} (1 ≤ v_{i} ≤ 10^9), where v_{i} is the contrast of the i-th photo.
-----Output-----
Print the minimal processing time of the division into groups.
-----Examples-----
Input
5 2
50 110 130 40 120
Output
20
Input
4 1
2 3 4 1
Output
0
-----Note-----
In the first example the photos should be split into 2 groups: [40, 50] and [110, 120, 130]. The processing time of the first group is 10, and the processing time of the second group is 20. Maximum among 10 and 20 is 20. It is impossible to split the photos into groups in a such way that the processing time of division is less than 20.
In the second example the photos should be split into four groups, each containing one photo. So the minimal possible processing time of a division is 0. | def f(m):
nonlocal dp, sdp
l = 0
for i in range(n):
while l < n and v[l] < v[i] - m:
l += 1
if l - 1 > i - k:
dp[i] = False
else:
dp[i] = (sdp[i - k + 1] != sdp[l - 1])
sdp[i + 1] = sdp[i] + (1 if dp[i] else 0)
return dp[n - 1]
n, k = list(map(int, input().split()))
dp = [False for i in range(n + 2)]
sdp = [0 for i in range(n + 2)]
dp[-1] = True
sdp[0] = 1
v = list(map(int, input().split()))
v.sort()
le = -1
r = v[-1] - v[0]
while r - le > 1:
m = (r + le) // 2
if f(m):
r = m
else:
le = m
print(r)
| def f(m):
nonlocal dp, sdp
l = 0
for i in range(n):
while l < n and v[l] < v[i] - m:
l += 1
if l - 1 > i - k:
dp[i] = False
else:
dp[i] = (sdp[i - k + 1] != sdp[l - 1])
sdp[i + 1] = sdp[i] + (1 if dp[i] else 0)
return dp[n - 1]
n, k = list(map(int, input().split()))
dp = [False for i in range(n + 2)]
sdp = [0 for i in range(n + 2)]
dp[-1] = True
sdp[0] = 1
v = list(map(int, input().split()))
v.sort()
le = -1
r = v[-1] - v[0]
while r - le > 1:
m = (r + le) // 2
if f(m):
r = m
else:
le = m
print(r)
| train | APPS_structured |
# Task
Given a sequence of 0s and 1s, determine if it is a prefix of Thue-Morse sequence.
The infinite Thue-Morse sequence is obtained by first taking a sequence containing a single 0 and then repeatedly concatenating the current sequence with its binary complement.
A binary complement of a sequence X is a sequence Y of the same length such that the sum of elements X_i and Y_i on the same positions is equal to 1 for each i.
Thus the first few iterations to obtain Thue-Morse sequence are:
```
0
0 1
0 1 1 0
0 1 1 0 1 0 0 1
...
```
# Examples
For `seq=[0, 1, 1, 0, 1]`, the result should be `true`.
For `seq=[0, 1, 0, 0]`, the result should be `false`.
# Inputs & Output
- `[input]` integer array `seq`
An non-empty array.
Constraints:
`1 <= seq.length <= 1000`
`seq[i] ∈ [0,1]`
- `[output]` a boolean value
`true` if it is a prefix of Thue-Morse sequence. `false` otherwise. | def is_thue_morse(seq):
init_seq = [0]
while len(init_seq) < len(seq):
init_seq += [1 if n == 0 else 0 for n in init_seq]
return init_seq[:len(seq)] == seq
| def is_thue_morse(seq):
init_seq = [0]
while len(init_seq) < len(seq):
init_seq += [1 if n == 0 else 0 for n in init_seq]
return init_seq[:len(seq)] == seq
| train | APPS_structured |
Rodriguez is a happy and content farmer. He has got a square field of side length $x$. Miguel, his son has himself grown into a man and his father wants to gift him something out of which he can make a living. So he gift's his son a square piece of land cut out from a corner of his field of side length $ y (y < x) $ leaving him with a L-shaped land.
But in Spanish tradition, it is considered inauspicious to own something which is prime in number. This worries Rodriguez as he doesn't want his left out area to be a prime number leading to bad luck. Find whether the spilt will be in terms with the tradition leaving Rodriguez happy.
-----Input:-----
- First line will contain $T$, number of testcases. Then the testcases follow.
- Each testcase contains two integers $x, y$.
-----Output:-----
Print YES if Rodriguez will be happy. Otherwise print NO.
-----Constraints-----
- $1 \leq T \leq 5$
- $1 \leq y < x \leq 100000000000$
-----Sample Input:-----
2
7 5
6 5
-----Sample Output:-----
YES
NO
-----EXPLANATION:-----
In case 1 :
Left out area is 24, which is not prime.
In case 2:
Left out area is 11, which is prime. | from math import sqrt
def isPrime(n):
for i in range(2, int(sqrt(n))+1):
if(n%i==0): return True
return False
ans = []
for _ in range(int(input())):
x, y = map(int, input().split())
ans.append('NO' if(isPrime(x**2-y**2)) else 'YES')
print('\n'.join(ans)) | from math import sqrt
def isPrime(n):
for i in range(2, int(sqrt(n))+1):
if(n%i==0): return True
return False
ans = []
for _ in range(int(input())):
x, y = map(int, input().split())
ans.append('NO' if(isPrime(x**2-y**2)) else 'YES')
print('\n'.join(ans)) | train | APPS_structured |
Given an array arr of positive integers sorted in a strictly increasing order, and an integer k.
Find the kth positive integer that is missing from this array.
Example 1:
Input: arr = [2,3,4,7,11], k = 5
Output: 9
Explanation: The missing positive integers are [1,5,6,8,9,10,12,13,...]. The 5th missing positive integer is 9.
Example 2:
Input: arr = [1,2,3,4], k = 2
Output: 6
Explanation: The missing positive integers are [5,6,7,...]. The 2nd missing positive integer is 6.
Constraints:
1 <= arr.length <= 1000
1 <= arr[i] <= 1000
1 <= k <= 1000
arr[i] < arr[j] for 1 <= i < j <= arr.length | class Solution:
def findKthPositive(self, arr: List[int], k: int) -> int:
n_miss = 0
i = 0
c = 1
n = len(arr)
while n_miss < k:
if i < n and arr[i] == c:
c += 1
i += 1
else:
c += 1
n_miss += 1
return c - 1 | class Solution:
def findKthPositive(self, arr: List[int], k: int) -> int:
n_miss = 0
i = 0
c = 1
n = len(arr)
while n_miss < k:
if i < n and arr[i] == c:
c += 1
i += 1
else:
c += 1
n_miss += 1
return c - 1 | train | APPS_structured |
Chef likes all arrays equally. But he likes some arrays more equally than others. In particular, he loves Rainbow Arrays.
An array is Rainbow if it has the following structure:
- First a1 elements equal 1.
- Next a2 elements equal 2.
- Next a3 elements equal 3.
- Next a4 elements equal 4.
- Next a5 elements equal 5.
- Next a6 elements equal 6.
- Next a7 elements equal 7.
- Next a6 elements equal 6.
- Next a5 elements equal 5.
- Next a4 elements equal 4.
- Next a3 elements equal 3.
- Next a2 elements equal 2.
- Next a1 elements equal 1.
- ai can be any non-zero positive integer.
- There are no other elements in array.
Help Chef in finding out if the given array is a Rainbow Array or not.
-----Input-----
- The first line of the input contains an integer T denoting the number of test cases.
- The first line of each test case contains an integer N, denoting the number of elements in the given array.
- The second line contains N space-separated integers A1, A2, ..., AN denoting the elements of array.
-----Output-----
- For each test case, output a line containing "yes" or "no" (without quotes) corresponding to the case if the array is rainbow array or not.
-----Constraints-----
- 1 ≤ T ≤ 100
- 7 ≤ N ≤ 100
- 1 ≤ Ai ≤ 10
-----Subtasks-----
- Subtask 1 (100 points) : Original constraints
-----Example-----
Input
3
19
1 2 3 4 4 5 6 6 6 7 6 6 6 5 4 4 3 2 1
14
1 2 3 4 5 6 7 6 5 4 3 2 1 1
13
1 2 3 4 5 6 8 6 5 4 3 2 1
Output
yes
no
no
-----Explanation-----
The first example satisfies all the conditions.
The second example has 1 element of value 1 at the beginning and 2 elements of value 1 at the end.
The third one has no elements with value 7 after elements with value 6. | t = int(input())
for i in range(t):
l = int(input())
li = list(map(int, input().split()))[:l]
a = []
b = [1, 2, 3, 4, 5, 6, 7]
c = li[::-1]
for i in li:
if i not in a:
a.append(i)
a.sort()
if a == b and li == c:
print('yes')
else:
print('no') | t = int(input())
for i in range(t):
l = int(input())
li = list(map(int, input().split()))[:l]
a = []
b = [1, 2, 3, 4, 5, 6, 7]
c = li[::-1]
for i in li:
if i not in a:
a.append(i)
a.sort()
if a == b and li == c:
print('yes')
else:
print('no') | train | APPS_structured |
Given an array of integers nums and an integer target.
Return the number of non-empty subsequences of nums such that the sum of the minimum and maximum element on it is less or equal than target.
Since the answer may be too large, return it modulo 10^9 + 7.
Example 1:
Input: nums = [3,5,6,7], target = 9
Output: 4
Explanation: There are 4 subsequences that satisfy the condition.
[3] -> Min value + max value <= target (3 + 3 <= 9)
[3,5] -> (3 + 5 <= 9)
[3,5,6] -> (3 + 6 <= 9)
[3,6] -> (3 + 6 <= 9)
Example 2:
Input: nums = [3,3,6,8], target = 10
Output: 6
Explanation: There are 6 subsequences that satisfy the condition. (nums can have repeated numbers).
[3] , [3] , [3,3], [3,6] , [3,6] , [3,3,6]
Example 3:
Input: nums = [2,3,3,4,6,7], target = 12
Output: 61
Explanation: There are 63 non-empty subsequences, two of them don't satisfy the condition ([6,7], [7]).
Number of valid subsequences (63 - 2 = 61).
Example 4:
Input: nums = [5,2,4,1,7,6,8], target = 16
Output: 127
Explanation: All non-empty subset satisfy the condition (2^7 - 1) = 127
Constraints:
1 <= nums.length <= 10^5
1 <= nums[i] <= 10^6
1 <= target <= 10^6 | class Solution:
#Version 1: Sliding window
#Count the mismatch count
#TC: O(nlogn), SC: O(1)
'''
def numSubseq(self, nums: List[int], target: int) -> int:
M = 10**9 + 7
mismatch = 0
nums.sort()
base = 0
for k in range(len(nums)):
if nums[k]*2 > target:
while base > 0 and nums[base] + nums[k] > target:
base -= 1
while base < k and nums[base] + nums[k] <= target:
base += 1
mismatch += pow(2, k-base, M)
return (pow(2, len(nums), M) - 1 - mismatch) % M
'''
#Version 2: Two sum
#Once the window is valid, we can add the number of subsequences with left or right number
#TC: O(nlogn), SC: O(1)
'''
def numSubseq(self, nums: List[int], target: int) -> int:
M = 10**9 + 7
pows = [1]*(len(nums)+1)
for k in range(1, len(pows)):
pows[k] = (pows[k-1]<<1) % M
ans = 0
nums.sort()
left = 0
right = len(nums) - 1
while left <= right:
total = nums[left] + nums[right]
if total > target:
right -= 1
else:
ans = (ans + pows[right-left]) % M
left += 1
return ans
'''
#Version 3: Sliding window
#Consider the valid count
#TC: O(n^2), SC: O(1)
def numSubseq(self, nums: List[int], target: int) -> int:
from bisect import bisect_left
M = 10**9 + 7
pows = [1]*(len(nums)+1)
for k in range(1, len(pows)):
pows[k] = (pows[k-1]<<1) % M
ans = 0
nums.sort()
base = 0
for k in range(len(nums)):
if nums[k]*2 <= target:
ans += pow(2, k, M)
else:
base = bisect_left(nums, target - nums[k] + 1)
ans += (pow(2, base, M) - 1) * pow(2, k-base, M)
#print(k, base, ans)
return ans % M
| class Solution:
#Version 1: Sliding window
#Count the mismatch count
#TC: O(nlogn), SC: O(1)
'''
def numSubseq(self, nums: List[int], target: int) -> int:
M = 10**9 + 7
mismatch = 0
nums.sort()
base = 0
for k in range(len(nums)):
if nums[k]*2 > target:
while base > 0 and nums[base] + nums[k] > target:
base -= 1
while base < k and nums[base] + nums[k] <= target:
base += 1
mismatch += pow(2, k-base, M)
return (pow(2, len(nums), M) - 1 - mismatch) % M
'''
#Version 2: Two sum
#Once the window is valid, we can add the number of subsequences with left or right number
#TC: O(nlogn), SC: O(1)
'''
def numSubseq(self, nums: List[int], target: int) -> int:
M = 10**9 + 7
pows = [1]*(len(nums)+1)
for k in range(1, len(pows)):
pows[k] = (pows[k-1]<<1) % M
ans = 0
nums.sort()
left = 0
right = len(nums) - 1
while left <= right:
total = nums[left] + nums[right]
if total > target:
right -= 1
else:
ans = (ans + pows[right-left]) % M
left += 1
return ans
'''
#Version 3: Sliding window
#Consider the valid count
#TC: O(n^2), SC: O(1)
def numSubseq(self, nums: List[int], target: int) -> int:
from bisect import bisect_left
M = 10**9 + 7
pows = [1]*(len(nums)+1)
for k in range(1, len(pows)):
pows[k] = (pows[k-1]<<1) % M
ans = 0
nums.sort()
base = 0
for k in range(len(nums)):
if nums[k]*2 <= target:
ans += pow(2, k, M)
else:
base = bisect_left(nums, target - nums[k] + 1)
ans += (pow(2, base, M) - 1) * pow(2, k-base, M)
#print(k, base, ans)
return ans % M
| train | APPS_structured |
One way to serialize a binary tree is to use pre-order traversal. When we encounter a non-null node, we record the node's value. If it is a null node, we record using a sentinel value such as #.
_9_
/ \
3 2
/ \ / \
4 1 # 6
/ \ / \ / \
# # # # # #
For example, the above binary tree can be serialized to the string "9,3,4,#,#,1,#,#,2,#,6,#,#", where # represents a null node.
Given a string of comma separated values, verify whether it is a correct preorder traversal serialization of a binary tree. Find an algorithm without reconstructing the tree.
Each comma separated value in the string must be either an integer or a character '#' representing null pointer.
You may assume that the input format is always valid, for example it could never contain two consecutive commas such as "1,,3".
Example 1:
Input: "9,3,4,#,#,1,#,#,2,#,6,#,#"
Output: true
Example 2:
Input: "1,#"
Output: false
Example 3:
Input: "9,#,#,1"
Output: false | class Solution:
def isValidSerialization(self, preorder):
"""
:type preorder: str
:rtype: bool
"""
arr = preorder.split(',')
k = len(arr)
for _ in range(k):
print(arr)
if arr==['#']:
return True
newArr = []
i = 0
while i < len(arr):
if i < len(arr)-2 and arr[i].isdigit() and arr[i+1:i+3] == ['#','#']:
newArr += ['#']
i += 3
else:
newArr += [arr[i]]
i += 1
arr = newArr
return False
| class Solution:
def isValidSerialization(self, preorder):
"""
:type preorder: str
:rtype: bool
"""
arr = preorder.split(',')
k = len(arr)
for _ in range(k):
print(arr)
if arr==['#']:
return True
newArr = []
i = 0
while i < len(arr):
if i < len(arr)-2 and arr[i].isdigit() and arr[i+1:i+3] == ['#','#']:
newArr += ['#']
i += 3
else:
newArr += [arr[i]]
i += 1
arr = newArr
return False
| train | APPS_structured |
=====Function Descriptions=====
zip([iterable, ...])
This function returns a list of tuples. The ith tuple contains the ith element from each of the argument sequences or iterables. If the argument sequences are of unequal lengths, then the returned list is truncated to the length of the shortest argument sequence.
Sample Code
>>> print zip([1,2,3,4,5,6],'Hacker')
[(1, 'H'), (2, 'a'), (3, 'c'), (4, 'k'), (5, 'e'), (6, 'r')]
>>>
>>> print zip([1,2,3,4,5,6],[0,9,8,7,6,5,4,3,2,1])
[(1, 0), (2, 9), (3, 8), (4, 7), (5, 6), (6, 5)]
>>>
>>> A = [1,2,3]
>>> B = [6,5,4]
>>> C = [7,8,9]
>>> X = [A] + [B] + [C]
>>>
>>> print zip(*X)
[(1, 6, 7), (2, 5, 8), (3, 4, 9)]
=====Problem Statement=====
The National University conducts an examination of N students in X subjects.
Your task is to compute the average scores of each student.
Average score = Sum of scores obtained in all subjects by a student / Total number of subjects
The format for the general mark sheet is:
Student ID -> ___1_____2_____3_____4_____5__
Subject 1 | 89 90 78 93 80
Subject 2 | 90 91 85 88 86
Subject 3 | 91 92 83 89 90.5
|______________________________
Average 90 91 82 90 85.5
=====Input Format=====
The first line contains N and X separated by a space. The next X lines contains the space separated marks obtained by students in a particular subject.
=====Constraints=====
0<N≤100
0<X≤100
=====Output Format=====
Print the averages of all students on separate lines. The averages must be correct up to 1 decimal place. | n, x = list(map(int,input().split()))
ar = [0 for i in range(n)]
for i in range(x):
temp_ar=list(map(float,input().split()))
for j in range(n):
ar[j] += temp_ar[j]
for i in range(n):
print((ar[i]/x))
| n, x = list(map(int,input().split()))
ar = [0 for i in range(n)]
for i in range(x):
temp_ar=list(map(float,input().split()))
for j in range(n):
ar[j] += temp_ar[j]
for i in range(n):
print((ar[i]/x))
| train | APPS_structured |
# Task
You are given three integers `l, d and x`. Your task is:
```
• determine the minimal integer n
such that l ≤ n ≤ d, and the sum of its digits equals x.
• determine the maximal integer m
such that l ≤ m ≤ d, and the sum of its digits equals x.
```
It is guaranteed that such numbers always exist.
# Input/Output
- `[input]` integer `l`
- `[input]` integer `d`
`1 ≤ l ≤ d ≤ 10000.`
- `[input]` integer `x`
`1 ≤ x ≤ 36`
- `[output]` an integer array
Array of two elements, where the first element is `n`, and the second one is `m`.
# Example
For `l = 500, d = 505, x = 10`, the output should be `[505, 505]`.
For `l = 100, d = 200, x = 10`, the output should be `[109, 190]`. | def min_and_max(l, d, x):
for n in range(l, d+1):
if sum(map(int, str(n))) == x: break
for m in range(d, l-1, -1):
if sum(map(int, str(m))) == x: break
return [n, m] | def min_and_max(l, d, x):
for n in range(l, d+1):
if sum(map(int, str(n))) == x: break
for m in range(d, l-1, -1):
if sum(map(int, str(m))) == x: break
return [n, m] | train | APPS_structured |
Given an integer `n` return `"odd"` if the number of its divisors is odd. Otherwise return `"even"`.
**Note**: big inputs will be tested.
## Examples:
All prime numbers have exactly two divisors (hence `"even"`).
For `n = 12` the divisors are `[1, 2, 3, 4, 6, 12]` – `"even"`.
For `n = 4` the divisors are `[1, 2, 4]` – `"odd"`. | oddity=lambda n: ["odd","even"][n**.5%1!=0] | oddity=lambda n: ["odd","even"][n**.5%1!=0] | train | APPS_structured |
Student A and student B are giving each other test answers during a test.
They don't want to be caught so they are sending each other coded messages.
Student A is sending student B the message: `Answer to Number 5 Part b`.
He starts of with a square grid (in this example the grid = 5x5).
He writes the message down (with spaces):
```
Answe
r to
Numbe
r 5 P
art b
```
He then starts writing the message down one column at a time (from the top to the bottom).
The new message is now: `ArNran u rstm5twob e ePb`
You are the teacher of this class.
Your job is to decipher this message and bust the students.
# Task
Write a function `decipher_message`.
This function will take one parameter (`message`).
This function will return the original message.
*** Note: The length of the string is always going to be a prefect square ***
Hint: You should probably decipher the example message first before you start coding
Have fun !!! | import math
def decipher_message(m):
l = int(math.sqrt(len(m)))
return ''.join(''.join(m[i+l*j] for j in range(l)) for i in range(l)) | import math
def decipher_message(m):
l = int(math.sqrt(len(m)))
return ''.join(''.join(m[i+l*j] for j in range(l)) for i in range(l)) | train | APPS_structured |
Let N be a positive even number.
We have a permutation of (1, 2, ..., N), p = (p_1, p_2, ..., p_N).
Snuke is constructing another permutation of (1, 2, ..., N), q, following the procedure below.
First, let q be an empty sequence.
Then, perform the following operation until p becomes empty:
- Select two adjacent elements in p, and call them x and y in order. Remove x and y from p (reducing the length of p by 2), and insert x and y, preserving the original order, at the beginning of q.
When p becomes empty, q will be a permutation of (1, 2, ..., N).
Find the lexicographically smallest permutation that can be obtained as q.
-----Constraints-----
- N is an even number.
- 2 ≤ N ≤ 2 × 10^5
- p is a permutation of (1, 2, ..., N).
-----Input-----
Input is given from Standard Input in the following format:
N
p_1 p_2 ... p_N
-----Output-----
Print the lexicographically smallest permutation, with spaces in between.
-----Sample Input-----
4
3 2 4 1
-----Sample Output-----
3 1 2 4
The solution above is obtained as follows:pq(3, 2, 4, 1)()↓↓(3, 1)(2, 4)↓↓()(3, 1, 2, 4) | from heapq import heappop, heappush
n = int(input())
p = [int(x) for x in input().split()]
class SegmentTree: # 0-indexed
def __init__(self, array, operation=min, identity=10**30):
self.identity = identity
self.n = len(array)
self.N = 1 << (self.n - 1).bit_length()
self.tree = [self.identity] * 2 * self.N
self.opr = operation
for i in range(self.n):
self.tree[i+self.N-1] = array[i]
for i in range(self.N-2, -1, -1):
self.tree[i] = self.opr(self.tree[2*i+1], self.tree[2*i+2])
def values(self):
return self.tree[self.N-1:]
def update(self, k, x):
k += self.N-1
self.tree[k] = x
while k+1:
k = (k-1)//2
self.tree[k] = self.opr(self.tree[k*2+1], self.tree[k*2+2])
def query(self, p, q): # [p,q)
if q <= p:
print("Oops! That was no valid number. Try again...")
return
p += self.N-1
q += self.N-2
res = self.identity
while q-p > 1:
if p & 1 == 0:
res = self.opr(res, self.tree[p])
if q & 1 == 1:
res = self.opr(res, self.tree[q])
q -= 1
p = p//2
q = (q-1)//2
if p == q:
res = self.opr(res, self.tree[p])
else:
res = self.opr(self.opr(res, self.tree[p]), self.tree[q])
return res
ind = [0]*(n+1)
Odd = SegmentTree([10**30]*n)
Even = SegmentTree([10**30]*n)
for i in range(n):
ind[p[i]] = i
if i % 2 == 0:
Even.update(i, p[i])
else:
Odd.update(i, p[i])
cand = []
heappush(cand, (Even.query(0, n), 0, n, True))
q = []
while len(q) < n:
first, l, r, is_even = heappop(cand)
if is_even:
second = Odd.query(ind[first]+1, r)
q.extend([first, second])
if l < ind[first]:
heappush(cand, (Even.query(l, ind[first]), l, ind[first], True))
if ind[first] + 1 < ind[second]:
heappush(
cand, (Odd.query(ind[first]+1, ind[second]), ind[first]+1, ind[second], False))
if ind[second]+1 < r:
heappush(
cand, (Even.query(ind[second], r), ind[second]+1, r, True))
else:
second = Even.query(ind[first]+1, r)
q.extend([first, second])
if l < ind[first]:
heappush(cand, (Odd.query(l, ind[first]), l, ind[first], False))
if ind[first] + 1 < ind[second]:
heappush(
cand, (Even.query(ind[first]+1, ind[second]), ind[first]+1, ind[second], True))
if ind[second]+1 < r:
heappush(
cand, (Odd.query(ind[second], r), ind[second]+1, r, False))
print((*q))
| from heapq import heappop, heappush
n = int(input())
p = [int(x) for x in input().split()]
class SegmentTree: # 0-indexed
def __init__(self, array, operation=min, identity=10**30):
self.identity = identity
self.n = len(array)
self.N = 1 << (self.n - 1).bit_length()
self.tree = [self.identity] * 2 * self.N
self.opr = operation
for i in range(self.n):
self.tree[i+self.N-1] = array[i]
for i in range(self.N-2, -1, -1):
self.tree[i] = self.opr(self.tree[2*i+1], self.tree[2*i+2])
def values(self):
return self.tree[self.N-1:]
def update(self, k, x):
k += self.N-1
self.tree[k] = x
while k+1:
k = (k-1)//2
self.tree[k] = self.opr(self.tree[k*2+1], self.tree[k*2+2])
def query(self, p, q): # [p,q)
if q <= p:
print("Oops! That was no valid number. Try again...")
return
p += self.N-1
q += self.N-2
res = self.identity
while q-p > 1:
if p & 1 == 0:
res = self.opr(res, self.tree[p])
if q & 1 == 1:
res = self.opr(res, self.tree[q])
q -= 1
p = p//2
q = (q-1)//2
if p == q:
res = self.opr(res, self.tree[p])
else:
res = self.opr(self.opr(res, self.tree[p]), self.tree[q])
return res
ind = [0]*(n+1)
Odd = SegmentTree([10**30]*n)
Even = SegmentTree([10**30]*n)
for i in range(n):
ind[p[i]] = i
if i % 2 == 0:
Even.update(i, p[i])
else:
Odd.update(i, p[i])
cand = []
heappush(cand, (Even.query(0, n), 0, n, True))
q = []
while len(q) < n:
first, l, r, is_even = heappop(cand)
if is_even:
second = Odd.query(ind[first]+1, r)
q.extend([first, second])
if l < ind[first]:
heappush(cand, (Even.query(l, ind[first]), l, ind[first], True))
if ind[first] + 1 < ind[second]:
heappush(
cand, (Odd.query(ind[first]+1, ind[second]), ind[first]+1, ind[second], False))
if ind[second]+1 < r:
heappush(
cand, (Even.query(ind[second], r), ind[second]+1, r, True))
else:
second = Even.query(ind[first]+1, r)
q.extend([first, second])
if l < ind[first]:
heappush(cand, (Odd.query(l, ind[first]), l, ind[first], False))
if ind[first] + 1 < ind[second]:
heappush(
cand, (Even.query(ind[first]+1, ind[second]), ind[first]+1, ind[second], True))
if ind[second]+1 < r:
heappush(
cand, (Odd.query(ind[second], r), ind[second]+1, r, False))
print((*q))
| train | APPS_structured |
**The Rub**
You need to make a function that takes an object as an argument, and returns a very similar object but with a special property. The returned object should allow a user to access values by providing only the beginning of the key for the value they want. For example if the given object has a key `idNumber`, you should be able to access its value on the returned object by using a key `idNum` or even simply `id`. `Num` and `Number` shouldn't work because we are only looking for matches at the beginning of a key.
Be aware that you _could_ simply add all these partial keys one by one to the object. However, for the sake of avoiding clutter, we don't want to have a JSON with a bunch of nonsensical keys. Thus, in the random tests there will be a test to check that you did not add or remove any keys from the object passed in or the object returned.
Also, if a key is tested that appears as the beginning of more than one key in the original object (e.g. if the original object had a key `idNumber` and `idString` and we wanted to test the key `id`) then return the value corresponding with whichever key comes first **alphabetically**. (In this case it would be `idNumber`s value because it comes first alphabetically.)
**Example**
```python
o = partial_keys({"abcd": 1})
o['abcd'] == 1 # true
o['abc'] == 1 # true
o['ab'] == 1 # true
o['a'] == 1 # true
o['b'] == 1 # false!
o['b'] == None # true
list(o.keys()) # ['abcd']
``` | class partial_keys(dict): __getitem__ = lambda self, key: self.get(next((k for k in sorted(self.keys()) if k.startswith(key)), None), None) | class partial_keys(dict): __getitem__ = lambda self, key: self.get(next((k for k in sorted(self.keys()) if k.startswith(key)), None), None) | train | APPS_structured |
Given a list of 24-hour clock time points in "Hour:Minutes" format, find the minimum minutes difference between any two time points in the list.
Example 1:
Input: ["23:59","00:00"]
Output: 1
Note:
The number of time points in the given list is at least 2 and won't exceed 20000.
The input time is legal and ranges from 00:00 to 23:59. | class Solution:
def findMinDifference(self, timePoints):
"""
:type timePoints: List[str]
:rtype: int
"""
def cv2mnt(t):
h,m = t.split(':')
mnt = int(h) * 60 + int(m)
return mnt
lst = [0 for i in range(1440)]
for t in timePoints:
mnt = cv2mnt(t)
if lst[mnt] == 1:
return 0
else:
lst[mnt] = 1
beg = 0
stk = [i for i,n in enumerate(lst) if n == 1]
stk.append(stk[0] + 1440)
res = 1439
for i in range(len(stk) - 1):
dif = abs(stk[i] - stk[i+1])
dif_ = min(1440 - dif, dif)
res = min(res,dif_)
if res == 1:
return 1
return res
| class Solution:
def findMinDifference(self, timePoints):
"""
:type timePoints: List[str]
:rtype: int
"""
def cv2mnt(t):
h,m = t.split(':')
mnt = int(h) * 60 + int(m)
return mnt
lst = [0 for i in range(1440)]
for t in timePoints:
mnt = cv2mnt(t)
if lst[mnt] == 1:
return 0
else:
lst[mnt] = 1
beg = 0
stk = [i for i,n in enumerate(lst) if n == 1]
stk.append(stk[0] + 1440)
res = 1439
for i in range(len(stk) - 1):
dif = abs(stk[i] - stk[i+1])
dif_ = min(1440 - dif, dif)
res = min(res,dif_)
if res == 1:
return 1
return res
| train | APPS_structured |
The game of billiards involves two players knocking 3 balls around
on a green baize table. Well, there is more to it, but for our
purposes this is sufficient.
The game consists of several rounds and in each round both players
obtain a score, based on how well they played. Once all the rounds
have been played, the total score of each player is determined by
adding up the scores in all the rounds and the player with the higher
total score is declared the winner.
The Siruseri Sports Club organises an annual billiards game where
the top two players of Siruseri play against each other. The Manager
of Siruseri Sports Club decided to add his own twist to the game by
changing the rules for determining the winner. In his version, at the
end of each round, the cumulative score for each player is calculated, and the leader and her current lead are found. Once
all the rounds are over the player who had the maximum lead at the
end of any round in the game is declared the winner.
Consider the following score sheet for a game with 5 rounds:
RoundPlayer 1Player 2114082289134390110411210658890
The total scores of both players, the leader and the lead after
each round for this game is given below:RoundPlayer 1Player 2LeaderLead114082Player 1582229216Player 1133319326Player 274431432Player 215519522Player 23
Note that the above table contains the cumulative scores.
The winner of this game is Player 1 as he had the maximum lead (58
at the end of round 1) during the game.
Your task is to help the Manager find the winner and the winning
lead. You may assume that the scores will be such that there will
always be a single winner. That is, there are no ties.
Input
The first line of the input will contain a single integer N (N
≤ 10000) indicating the number of rounds in the game. Lines
2,3,...,N+1 describe the scores of the two players in the N rounds.
Line i+1 contains two integer Si and Ti, the scores of the Player 1
and 2 respectively, in round i. You may assume that 1 ≤ Si ≤
1000 and 1 ≤ Ti ≤ 1000.
Output
Your output must consist of a single line containing two integers
W and L, where W is 1 or 2 and indicates the winner and L is the
maximum lead attained by the winner.
Example
Input:
5
140 82
89 134
90 110
112 106
88 90
Output:
1 58 | # cook your dish here
try:
n1=int(input())
wina=winb=ma=mb=n=m=0
for i in range(n1):
a,b=list(map(int,input().split()))
n+=a
m+=b
if n>m and n-m>ma:
ma=n-m
elif n<m and m-n>mb:
mb=m-n
if ma>mb:
print('1',ma)
elif ma<mb:
print('2',mb)
except:pass
| # cook your dish here
try:
n1=int(input())
wina=winb=ma=mb=n=m=0
for i in range(n1):
a,b=list(map(int,input().split()))
n+=a
m+=b
if n>m and n-m>ma:
ma=n-m
elif n<m and m-n>mb:
mb=m-n
if ma>mb:
print('1',ma)
elif ma<mb:
print('2',mb)
except:pass
| train | APPS_structured |
For her next karate demonstration, Ada will break some bricks.
Ada stacked three bricks on top of each other. Initially, their widths (from top to bottom) are $W_1, W_2, W_3$.
Ada's strength is $S$. Whenever she hits a stack of bricks, consider the largest $k \ge 0$ such that the sum of widths of the topmost $k$ bricks does not exceed $S$; the topmost $k$ bricks break and are removed from the stack. Before each hit, Ada may also decide to reverse the current stack of bricks, with no cost.
Find the minimum number of hits Ada needs in order to break all bricks if she performs the reversals optimally. You are not required to minimise the number of reversals.
-----Input-----
- The first line of the input contains a single integer $T$ denoting the number of test cases. The description of $T$ test cases follows.
- The first and only line of each test case contains four space-separated integers $S$, $W_1$, $W_2$ and $W_3$.
-----Output-----
For each test case, print a single line containing one integer ― the minimum required number of hits.
-----Constraints-----
- $1 \le T \le 64$
- $1 \le S \le 8$
- $1 \le W_i \le 2$ for each valid $i$
- it is guaranteed that Ada can break all bricks
-----Subtasks-----
Subtask #1 (50 points): $W_1 = W_2 = W_3$
Subtask #2 (50 points): original constraints
-----Example Input-----
3
3 1 2 2
2 1 1 1
3 2 2 1
-----Example Output-----
2
2
2
-----Explanation-----
Example case 1: Ada can reverse the stack and then hit it two times. Before the first hit, the widths of bricks in the stack (from top to bottom) are $(2,2,1)$. After the first hit, the topmost brick breaks and the stack becomes $(2,1)$. The second hit breaks both remaining bricks.
In this particular case, it is also possible to hit the stack two times without reversing. Before the first hit, it is $(1, 2, 2)$. The first hit breaks the two bricks at the top (so the stack becomes $(2)$) and the second hit breaks the last brick. | # cook your dish here
import math
t=int(input())
while t>0:
s,a,b,c=map(int,input().split())
if s>=a+b+c:
print("1")
elif s>=a+b or s>=b+c:
print("2")
else:
print("3")
t-=1 | # cook your dish here
import math
t=int(input())
while t>0:
s,a,b,c=map(int,input().split())
if s>=a+b+c:
print("1")
elif s>=a+b or s>=b+c:
print("2")
else:
print("3")
t-=1 | train | APPS_structured |
## The Riddle
The King of a small country invites 1000 senators to his annual party. As a tradition, each senator brings the King a bottle of wine. Soon after, the Queen discovers that one of the senators is trying to assassinate the King by giving him a bottle of poisoned wine. Unfortunately, they do not know which senator, nor which bottle of wine is poisoned, and the poison is completely indiscernible.
However, the King has 10 lab rats. He decides to use them as taste testers to determine which bottle of wine contains the poison. The poison when taken has no effect on the rats, until exactly 24 hours later when the infected rats suddenly die. The King needs to determine which bottle of wine is poisoned by tomorrow, so that the festivities can continue as planned.
Hence he only has time for one round of testing, he decides that each rat tastes multiple bottles, according to a certain scheme.
## Your Task
You receive an array of integers (`0 to 9`), each of them is the number of a rat which died after tasting the wine bottles. Return the number of the bottle (`1..1000`) which is poisoned.
**Good Luck!**
*Hint: think of rats as a certain representation of the number of the bottle...* | def find(r):
return sum([pow(2,a) for a in r])
| def find(r):
return sum([pow(2,a) for a in r])
| train | APPS_structured |
Given an array of integers and an integer k, you need to find the total number of continuous subarrays whose sum equals to k.
Example 1:
Input:nums = [1,1,1], k = 2
Output: 2
Note:
The length of the array is in range [1, 20,000].
The range of numbers in the array is [-1000, 1000] and the range of the integer k is [-1e7, 1e7]. | class Solution:
def subarraySum(self, nums, k):
"""
:type nums: List[int]
:type k: int
:rtype: int
"""
l_nums = len(nums)
c = 0
state = dict()
state[0] = 1
sum = 0
for idx in range(0, l_nums):
sum += nums[idx]
if((sum - k) in state):
c += state[sum - k]
state[sum] = (state[sum] if sum in state else 0) + 1
return c
| class Solution:
def subarraySum(self, nums, k):
"""
:type nums: List[int]
:type k: int
:rtype: int
"""
l_nums = len(nums)
c = 0
state = dict()
state[0] = 1
sum = 0
for idx in range(0, l_nums):
sum += nums[idx]
if((sum - k) in state):
c += state[sum - k]
state[sum] = (state[sum] if sum in state else 0) + 1
return c
| train | APPS_structured |
=====Example=====
In Python, a string can be split on a delimiter.
Example:
>>> a = "this is a string"
>>> a = a.split(" ") # a is converted to a list of strings.
>>> print a
['this', 'is', 'a', 'string']
Joining a string is simple:
>>> a = "-".join(a)
>>> print a
this-is-a-string
=====Problem Statement=====
You are given a string. Split the string on a " " (space) delimiter and join using a - hyphen.
=====Input Format=====
The first line contains a string consisting of space separated words.
=====Output Format=====
Print the formatted string as explained above. | # Enter your code here. Read input from STDIN. Print output to STDOUT
print(("-").join(input().strip().split()))
| # Enter your code here. Read input from STDIN. Print output to STDOUT
print(("-").join(input().strip().split()))
| train | APPS_structured |
The chef is trying to solve some pattern problems, Chef wants your help to code it. Chef has one number K to form a new pattern. Help the chef to code this pattern problem.
-----Input:-----
- First-line will contain $T$, the number of test cases. Then the test cases follow.
- Each test case contains a single line of input, one integer $K$.
-----Output:-----
For each test case, output as the pattern.
-----Constraints-----
- $1 \leq T \leq 100$
- $1 \leq K \leq 100$
-----Sample Input:-----
3
2
3
4
-----Sample Output:-----
21
1
123
21
1
4321
123
21
1
-----EXPLANATION:-----
No need, else pattern can be decode easily. | for _ in range(int(input())):
n = int(input())
l = list(range(1, n+1))
while l:
if len(l) % 2 == 0:
print(*l[::-1], sep='')
else:
print(*l, sep='')
l.pop() | for _ in range(int(input())):
n = int(input())
l = list(range(1, n+1))
while l:
if len(l) % 2 == 0:
print(*l[::-1], sep='')
else:
print(*l, sep='')
l.pop() | train | APPS_structured |
You are given a square matrix $M$ with $N$ rows (numbered $1$ through $N$) and $N$ columns (numbered $1$ through $N$). Initially, all the elements of this matrix are equal to $A$. The matrix is broken down in $N$ steps (numbered $1$ through $N$); note that during this process, some elements of the matrix are simply marked as removed, but all elements are still indexed in the same way as in the original matrix. For each valid $i$, the $i$-th step consists of the following:
- Elements $M_{1, N-i+1}, M_{2, N-i+1}, \ldots, M_{i-1, N-i+1}$ are removed.
- Elements $M_{i, N-i+1}, M_{i, N-i+2}, \ldots, M_{i, N}$ are removed.
- Let's denote the product of all $2i-1$ elements removed in this step by $p_i$. Each of the remaining elements of the matrix (those which have not been removed yet) is multiplied by $p_i$.
Find the sum $p_1 + p_2 + p_3 + \ldots + p_N$. Since this number could be very large, compute it modulo $10^9+7$.
-----Input-----
- The first line of the input contains a single integer $T$ denoting the number of test cases. The description of $T$ test cases follows.
- The first and only line of each test case contains two space-separated integers $N$ and $A$.
-----Output-----
For each test case, print a single line containing one integer ― the sum of products at each step modulo $10^9+7$.
-----Constraints-----
- $1 \le T \le 250$
- $1 \le N \le 10^5$
- $0 \le A \le 10^9$
- the sum of $N$ over all test cases does not exceed $10^5$
-----Example Input-----
1
3 2
-----Example Output-----
511620149
-----Explanation-----
Example case 1: | def pingalapower(a,n,mod):
if n==1:
return a
x=pingalapower(a,n//2,mod)
if n%2==0:
return (x*x)%mod
else:
return (x*x*a)%mod
for _ in range(int(input())):
n,a=map(int,input().split())
c=1
ans=0
mod=10**9+7
for i in range(n):
t_1=(pingalapower(a,c,mod)%mod)
ans=(ans+t_1)%mod
a=(a*t_1)%mod
c+=2
print(ans%mod) | def pingalapower(a,n,mod):
if n==1:
return a
x=pingalapower(a,n//2,mod)
if n%2==0:
return (x*x)%mod
else:
return (x*x*a)%mod
for _ in range(int(input())):
n,a=map(int,input().split())
c=1
ans=0
mod=10**9+7
for i in range(n):
t_1=(pingalapower(a,c,mod)%mod)
ans=(ans+t_1)%mod
a=(a*t_1)%mod
c+=2
print(ans%mod) | train | APPS_structured |
Alan's child can be annoying at times.
When Alan comes home and tells his kid what he has accomplished today, his kid never believes him.
Be that kid.
Your function 'AlanAnnoyingKid' takes as input a sentence spoken by Alan (a string). The sentence contains the following structure:
"Today I " + [action_verb] + [object] + "."
(e.g.: "Today I played football.")
Your function will return Alan's kid response, which is another sentence with the following structure:
"I don't think you " + [action_performed_by_alan] + " today, I think you " + ["did" OR "didn't"] + [verb_of _action_in_present_tense] + [" it!" OR " at all!"]
(e.g.:"I don't think you played football today, I think you didn't play at all!")
Note the different structure depending on the presence of a negation in Alan's first sentence (e.g., whether Alan says "I dind't play football", or "I played football").
! Also note: Alan's kid is young and only uses simple, regular verbs that use a simple "ed" to make past tense.
There are random test cases.
Some more examples:
input = "Today I played football."
output = "I don't think you played football today, I think you didn't play at all!"
input = "Today I didn't attempt to hardcode this Kata."
output = "I don't think you didn't attempt to hardcode this Kata today, I think you did attempt it!"
input = "Today I didn't play football."
output = "I don't think you didn't play football today, I think you did play it!"
input = "Today I cleaned the kitchen."
output = "I don't think you cleaned the kitchen today, I think you didn't clean at all!" | def alan_annoying_kid(stg):
s1 = stg.replace("Today I ", "")[:-1]
s3 = s1.replace("didn't ", "").split()[0]
s2, s3, s4 = ("did", s3, "it") if "n't" in s1 else ("didn't", s3[:-2], "at all")
return f"I don't think you {s1} today, I think you {s2} {s3} {s4}!" | def alan_annoying_kid(stg):
s1 = stg.replace("Today I ", "")[:-1]
s3 = s1.replace("didn't ", "").split()[0]
s2, s3, s4 = ("did", s3, "it") if "n't" in s1 else ("didn't", s3[:-2], "at all")
return f"I don't think you {s1} today, I think you {s2} {s3} {s4}!" | train | APPS_structured |
**See Also**
* [Traffic Lights - one car](.)
* [Traffic Lights - multiple cars](https://www.codewars.com/kata/5d230e119dd9860028167fa5)
---
# Overview
A character string represents a city road.
Cars travel on the road obeying the traffic lights..
Legend:
* `.` = Road
* `C` = Car
* `G` = GREEN traffic light
* `O` = ORANGE traffic light
* `R` = RED traffic light
Something like this:
C...R............G......
# Rules
## Simulation
At each iteration:
1. the lights change, according to the traffic light rules... then
2. the car moves, obeying the car rules
## Traffic Light Rules
Traffic lights change colour as follows:
* GREEN for `5` time units... then
* ORANGE for `1` time unit... then
* RED for `5` time units....
* ... and repeat the cycle
## Car Rules
* Cars travel left to right on the road, moving 1 character position per time unit
* Cars can move freely until they come to a traffic light. Then:
* if the light is GREEN they can move forward (temporarily occupying the same cell as the light)
* if the light is ORANGE then they must stop (if they have already entered the intersection they can continue through)
* if the light is RED the car must stop until the light turns GREEN again
# Kata Task
Given the initial state of the road, return the states for all iterations of the simiulation.
## Input
* `road` = the road array
* `n` = how many time units to simulate (n >= 0)
## Output
* An array containing the road states at every iteration (including the initial state)
* Note: If a car occupies the same position as a traffic light then show only the car
## Notes
* There is only one car
* For the initial road state
* the car is always at the first character position
* traffic lights are either GREEN or RED, and are at the beginning of their countdown cycles
* There are no reaction delays - when the lights change the car drivers will react immediately!
* If the car goes off the end of the road it just disappears from view
* There will always be some road between adjacent traffic lights
# Example
Run simulation for 10 time units
**Input**
* `road = "C...R............G......"`
* `n = 10`
**Result**
---
Good luck!
DM | from itertools import cycle
def traffic_lights(road, n):
light1 = iter(cycle( 'RRRRRGGGGGO' ))
light2 = iter(cycle( 'GGGGGORRRRR' ))
traffic , car = [], -1
for _ in range(n+1):
temp, RL, GL = [], next(light1), next(light2)
for i in range(len(road)):
temp.append( { 'R':RL, 'G':GL }.get(road[i], '.') )
if car<len(temp)-1 and temp[car+1] in 'RO': car -=1
car +=1
if car<len(temp): temp[car] = 'C'
traffic.append(''.join(temp))
return traffic | from itertools import cycle
def traffic_lights(road, n):
light1 = iter(cycle( 'RRRRRGGGGGO' ))
light2 = iter(cycle( 'GGGGGORRRRR' ))
traffic , car = [], -1
for _ in range(n+1):
temp, RL, GL = [], next(light1), next(light2)
for i in range(len(road)):
temp.append( { 'R':RL, 'G':GL }.get(road[i], '.') )
if car<len(temp)-1 and temp[car+1] in 'RO': car -=1
car +=1
if car<len(temp): temp[car] = 'C'
traffic.append(''.join(temp))
return traffic | train | APPS_structured |
Kshitij has recently started solving problems on codechef. As he is real problem solving enthusiast, he wants continuous growth in number of problems solved per day.
He started with $a$ problems on first day.
He solves $d$ problems more than previous day. But after every $k$ days , he increases $d$ by
$inc$ .
Can you guess how many questions he will solve on $nth $ day ?
-----Input:-----
- First line contains $T$, number of testcases. Then the testcases follow.
- Each testcase contains of a single line of input,five integers $a, d, k, n, inc$.
-----Output:-----
For each testcase, output in a single line number of questions solved on $nth$ day.
-----Constraints-----
- $1 \leq T \leq 15$
- $1 \leq a \leq 99$
- $1 \leq d \leq 100$
- $1 \leq n \leq 10000$
- $1 \leq k \leq n$
- $0 \leq inc \leq 99$
-----Sample Input:-----
1
1 4 3 8 2
-----Sample Output:-----
43
-----EXPLANATION:-----
The number of questions solved in first 8 days is :
$1$ $5$ $9$ $15$ $21$ $27$ $35$ $43$ .
On first day he solved 1 problem . Here $d$ is 4 for first 3 days.
Then after 3 days $d$ increases by 2 (that is 6). | try:
for _ in range(int(input())):
a,d,k,n,inc=map(int,input().split())
p=0
s=a
for i in range(n//k):
if i==0:
s+=(k-1)*d
d+=inc
else:
s+=k*d
d+=inc
s+=(n%k)*(d)
print(s)
except:
pass | try:
for _ in range(int(input())):
a,d,k,n,inc=map(int,input().split())
p=0
s=a
for i in range(n//k):
if i==0:
s+=(k-1)*d
d+=inc
else:
s+=k*d
d+=inc
s+=(n%k)*(d)
print(s)
except:
pass | train | APPS_structured |
I always thought that my old friend John was rather richer than he looked, but I never knew exactly how much money he actually had. One day (as I was plying him with questions) he said:
* "Imagine I have between `m` and `n` Zloty..." (or did he say Quetzal? I can't remember!)
* "If I were to buy **9** cars costing `c` each, I'd only have 1 Zloty (or was it Meticals?) left."
* "And if I were to buy **7** boats at `b` each, I'd only have 2 Ringglets (or was it Zloty?) left."
Could you tell me in each possible case:
1. how much money `f` he could possibly have ?
2. the cost `c` of a car?
3. the cost `b` of a boat?
So, I will have a better idea about his fortune. Note that if `m-n` is big enough, you might have a lot of possible answers.
Each answer should be given as `["M: f", "B: b", "C: c"]` and all the answers as `[ ["M: f", "B: b", "C: c"], ... ]`. "M" stands for money, "B" for boats, "C" for cars.
**Note:** `m, n, f, b, c` are positive integers, where `0 <= m <= n` or `m >= n >= 0`. `m` and `n` are inclusive.
## Examples:
```
howmuch(1, 100) => [["M: 37", "B: 5", "C: 4"], ["M: 100", "B: 14", "C: 11"]]
howmuch(1000, 1100) => [["M: 1045", "B: 149", "C: 116"]]
howmuch(10000, 9950) => [["M: 9991", "B: 1427", "C: 1110"]]
howmuch(0, 200) => [["M: 37", "B: 5", "C: 4"], ["M: 100", "B: 14", "C: 11"], ["M: 163", "B: 23", "C: 18"]]
```
Explanation of the results for `howmuch(1, 100)`:
* In the first answer his possible fortune is **37**:
* so he can buy 7 boats each worth 5: `37 - 7 * 5 = 2`
* or he can buy 9 cars worth 4 each: `37 - 9 * 4 = 1`
* The second possible answer is **100**:
* he can buy 7 boats each worth 14: `100 - 7 * 14 = 2`
* or he can buy 9 cars worth 11: `100 - 9 * 11 = 1`
# Note
See "Sample Tests" to know the format of the return. | howmuch=lambda m,n:[[f"M: {M}",f"B: {M//7}",f"C: {M//9}"]for M in range(min(m,n),max(m,n)+1)if M%7==2and M%9==1] | howmuch=lambda m,n:[[f"M: {M}",f"B: {M//7}",f"C: {M//9}"]for M in range(min(m,n),max(m,n)+1)if M%7==2and M%9==1] | train | APPS_structured |
Implement a function called makeAcronym that returns the first letters of each word in a passed in string.
Make sure the letters returned are uppercase.
If the value passed in is not a string return 'Not a string'.
If the value passed in is a string which contains characters other than spaces and alphabet letters, return 'Not letters'.
If the string is empty, just return the string itself: "".
**EXAMPLES:**
```
'Hello codewarrior' -> 'HC'
'a42' -> 'Not letters'
42 -> 'Not a string'
[2,12] -> 'Not a string'
{name: 'Abraham'} -> 'Not a string'
``` | def make_acronym(phrase):
if not isinstance(phrase, str): return 'Not a string'
arr = phrase.split()
return ''.join(a[0] for a in arr).upper() if all(map(str.isalpha, arr)) else 'Not letters' | def make_acronym(phrase):
if not isinstance(phrase, str): return 'Not a string'
arr = phrase.split()
return ''.join(a[0] for a in arr).upper() if all(map(str.isalpha, arr)) else 'Not letters' | train | APPS_structured |
Write a function that takes a list of at least four elements as an argument and returns a list of the middle two or three elements in reverse order. | def reverse_middle(lst):
l = len(lst)
return lst[(l+1)//2:(l//2)-2:-1] | def reverse_middle(lst):
l = len(lst)
return lst[(l+1)//2:(l//2)-2:-1] | train | APPS_structured |
Given an array of strings names of size n. You will create n folders in your file system such that, at the ith minute, you will create a folder with the name names[i].
Since two files cannot have the same name, if you enter a folder name which is previously used, the system will have a suffix addition to its name in the form of (k), where, k is the smallest positive integer such that the obtained name remains unique.
Return an array of strings of length n where ans[i] is the actual name the system will assign to the ith folder when you create it.
Example 1:
Input: names = ["pes","fifa","gta","pes(2019)"]
Output: ["pes","fifa","gta","pes(2019)"]
Explanation: Let's see how the file system creates folder names:
"pes" --> not assigned before, remains "pes"
"fifa" --> not assigned before, remains "fifa"
"gta" --> not assigned before, remains "gta"
"pes(2019)" --> not assigned before, remains "pes(2019)"
Example 2:
Input: names = ["gta","gta(1)","gta","avalon"]
Output: ["gta","gta(1)","gta(2)","avalon"]
Explanation: Let's see how the file system creates folder names:
"gta" --> not assigned before, remains "gta"
"gta(1)" --> not assigned before, remains "gta(1)"
"gta" --> the name is reserved, system adds (k), since "gta(1)" is also reserved, systems put k = 2. it becomes "gta(2)"
"avalon" --> not assigned before, remains "avalon"
Example 3:
Input: names = ["onepiece","onepiece(1)","onepiece(2)","onepiece(3)","onepiece"]
Output: ["onepiece","onepiece(1)","onepiece(2)","onepiece(3)","onepiece(4)"]
Explanation: When the last folder is created, the smallest positive valid k is 4, and it becomes "onepiece(4)".
Example 4:
Input: names = ["wano","wano","wano","wano"]
Output: ["wano","wano(1)","wano(2)","wano(3)"]
Explanation: Just increase the value of k each time you create folder "wano".
Example 5:
Input: names = ["kaido","kaido(1)","kaido","kaido(1)"]
Output: ["kaido","kaido(1)","kaido(2)","kaido(1)(1)"]
Explanation: Please note that system adds the suffix (k) to current name even it contained the same suffix before.
Constraints:
1 <= names.length <= 5 * 10^4
1 <= names[i].length <= 20
names[i] consists of lower case English letters, digits and/or round brackets. | class Solution:
def getFolderNames(self, names: List[str]) -> List[str]:
#cases: name doesn't exist just add
#name exists, add number to end, check for other instances
#name has number at end, add number to end so two numbers, dont handle as special? just treat as normal
#if name exists add number if that exists increment number
#if name(1) exists add number, if that exists increment number
#have dictionary with array of num values associated with it
ans, suffixNum = [], Counter()
for name in names:
if name in suffixNum:
while name + '(' + str(suffixNum[name]) + ')' in suffixNum:
suffixNum[name] += 1
ans.append(name + '(' + str(suffixNum[name]) + ')')
else:
ans.append(name)
suffixNum[ans[-1]] += 1
return ans
| class Solution:
def getFolderNames(self, names: List[str]) -> List[str]:
#cases: name doesn't exist just add
#name exists, add number to end, check for other instances
#name has number at end, add number to end so two numbers, dont handle as special? just treat as normal
#if name exists add number if that exists increment number
#if name(1) exists add number, if that exists increment number
#have dictionary with array of num values associated with it
ans, suffixNum = [], Counter()
for name in names:
if name in suffixNum:
while name + '(' + str(suffixNum[name]) + ')' in suffixNum:
suffixNum[name] += 1
ans.append(name + '(' + str(suffixNum[name]) + ')')
else:
ans.append(name)
suffixNum[ans[-1]] += 1
return ans
| train | APPS_structured |
Given the strings s1 and s2 of size n, and the string evil. Return the number of good strings.
A good string has size n, it is alphabetically greater than or equal to s1, it is alphabetically smaller than or equal to s2, and it does not contain the string evil as a substring. Since the answer can be a huge number, return this modulo 10^9 + 7.
Example 1:
Input: n = 2, s1 = "aa", s2 = "da", evil = "b"
Output: 51
Explanation: There are 25 good strings starting with 'a': "aa","ac","ad",...,"az". Then there are 25 good strings starting with 'c': "ca","cc","cd",...,"cz" and finally there is one good string starting with 'd': "da".
Example 2:
Input: n = 8, s1 = "leetcode", s2 = "leetgoes", evil = "leet"
Output: 0
Explanation: All strings greater than or equal to s1 and smaller than or equal to s2 start with the prefix "leet", therefore, there is not any good string.
Example 3:
Input: n = 2, s1 = "gx", s2 = "gz", evil = "x"
Output: 2
Constraints:
s1.length == n
s2.length == n
s1 <= s2
1 <= n <= 500
1 <= evil.length <= 50
All strings consist of lowercase English letters. | class Solution:
def findGoodStrings(self, n: int, s1: str, s2: str, evil: str) -> int:
M = 10**9 + 7
table = [-1]
pos, cnd = 1, 0
while pos < len(evil):
if evil[pos] == evil[cnd]:
table.append(table[cnd])
else:
table.append(cnd)
cnd = table[cnd]
while cnd >= 0 and evil[pos] != evil[cnd]:
cnd = table[cnd]
pos += 1
cnd += 1
table.append(cnd)
@lru_cache(None)
def dfs(i, j, u, b):
if j == len(evil):
return 0
if i == n:
return 1
r = 0
for c in range(ord(s1[i]) if b else 97, ord(s2[i])+1 if u else 123):
if c == ord(evil[j]):
t = dfs(i+1, j+1, u and c == ord(s2[i]), b and c == ord(s1[i]))
else:
p = j
while p >= 0:
if ord(evil[p]) == c:
break
p = table[p]
t = dfs(i+1, p+1, u and c == ord(s2[i]), b and c == ord(s1[i]))
r += t
return r % M
return dfs(0, 0, True, True) | class Solution:
def findGoodStrings(self, n: int, s1: str, s2: str, evil: str) -> int:
M = 10**9 + 7
table = [-1]
pos, cnd = 1, 0
while pos < len(evil):
if evil[pos] == evil[cnd]:
table.append(table[cnd])
else:
table.append(cnd)
cnd = table[cnd]
while cnd >= 0 and evil[pos] != evil[cnd]:
cnd = table[cnd]
pos += 1
cnd += 1
table.append(cnd)
@lru_cache(None)
def dfs(i, j, u, b):
if j == len(evil):
return 0
if i == n:
return 1
r = 0
for c in range(ord(s1[i]) if b else 97, ord(s2[i])+1 if u else 123):
if c == ord(evil[j]):
t = dfs(i+1, j+1, u and c == ord(s2[i]), b and c == ord(s1[i]))
else:
p = j
while p >= 0:
if ord(evil[p]) == c:
break
p = table[p]
t = dfs(i+1, p+1, u and c == ord(s2[i]), b and c == ord(s1[i]))
r += t
return r % M
return dfs(0, 0, True, True) | train | APPS_structured |
Given a certain square matrix ```A```, of dimension ```n x n```, that has negative and positive values (many of them may be 0).
We need the following values rounded to the closest integer:
- the average of all the positive numbers (more or equal to 0) that are in the principal diagonal and in the columns with odd index, **avg1**
- the absolute value of the average of all the negative numbers in the secondary diagonal and in the columns with even index, **avg2**
Let's see the requirements in an example:
```
A = [[ 1, 3, -4, 5, -2, 5, 1],
[ 2, 0, -7, 6, 8, 8, 15],
[ 4, 4, -2, -10, 7, -1, 7],
[ -1, 3, 1, 0, 11, 4, 21],
[ -7, 6, -4, 10, 5, 7, 6],
[ -5, 4, 3, -5, 7, 8, 17],
[-11, 3, 4, -8, 6, 16, 4]]
```
The elements of the principal diagonal are:
```
[1, 0, -2, 0, 5, 8, 4]
```
The ones that are also in the "odd columns" (we consider the index starting with 0) are:
```
[0, 0, 8] all positives
```
So,
```
avg1 = [8 / 3] = 3
```
The elements of the secondary diagonal are:
```
[-11, 4, -4, 0, 7, 8, 1]
```
The ones that are in the even columns are:
```
[-11, -4, 7, 1]
```
The negative ones are:
```
[-11, 4]
```
So,
```
avg2 = [|-15 / 2|] = 8
```
Create a function ```avg_diags()```that may receive a square matrix of uncertain dimensions and may output both averages in an array in the following order: ```[avg1, avg2]```
If one of the diagonals have no elements fulfilling the requirements described above the function should return ```-1```
So, if the function processes the matrix given above, it should output:
```
[3, 8]
```
Features of the random tests:
```
number of tests = 110
5 ≤ matrix_length ≤ 1000
-1000000 ≤ matrix[i, j] ≤ 1000000
```
Enjoy it!
Translations into another languages will be released soon. | import math
def avg_diags(m):
diagonal_1 = []
diagonal_2 = []
ans1 = 0
ans2 = 0
for i in range(len(m)):
if i %2 != 0 and m[i][i] >=0:
diagonal_1.append(m[i][i])
if i %2 == 0 and m[len(m)-1 -i][i] <0:
diagonal_2.append(m[len(m)-1 -i][i])
if diagonal_1 == []:
ans1 = -1
else:
ans1 =round(sum(diagonal_1)/len(diagonal_1),0)
if diagonal_2 == []:
ans2 = -1
else:
ans2 = round(sum(diagonal_2)*(-1)/len(diagonal_2),0)
return [ans1, ans2] | import math
def avg_diags(m):
diagonal_1 = []
diagonal_2 = []
ans1 = 0
ans2 = 0
for i in range(len(m)):
if i %2 != 0 and m[i][i] >=0:
diagonal_1.append(m[i][i])
if i %2 == 0 and m[len(m)-1 -i][i] <0:
diagonal_2.append(m[len(m)-1 -i][i])
if diagonal_1 == []:
ans1 = -1
else:
ans1 =round(sum(diagonal_1)/len(diagonal_1),0)
if diagonal_2 == []:
ans2 = -1
else:
ans2 = round(sum(diagonal_2)*(-1)/len(diagonal_2),0)
return [ans1, ans2] | train | APPS_structured |
Chef Ada has a matrix with $N$ rows (numbered $1$ through $N$ from top to bottom) and $N$ columns (numbered $1$ through $N$ from left to right) containing all integers between $1$ and $N^2$ inclusive. For each valid $i$ and $j$, let's denote the cell in the $i$-th row and $j$-th column by $(i,j)$.
Ada wants to sort the matrix in row-major order ― for each valid $i$ and $j$, she wants the cell $(i, j)$ to contain the value $(i-1) \cdot N + j$.
In one operation, Ada should choose an integer $L$ and transpose the submatrix between rows $1$ and $L$ and columns $1$ and $L$ (inclusive). Formally, for each $i$ and $j$ ($1 \le i, j \le L$), the value in the cell $(i, j)$ after this operation is equal to the value in $(j, i)$ before it.
The initial state of the matrix is chosen in such a way that it is possible to sort it using a finite number of operations (possibly zero). Find the smallest number of operations required to sort the matrix.
-----Input-----
- The first line of the input contains a single integer $T$ denoting the number of test cases. The description of $T$ test cases follows.
- The first line of each test case contains a single integer $N$.
- The next $N$ lines describe the matrix. For each valid $i$, the $i$-th of these lines contains $N$ space-separated integers ― the initial values in cells $(i, 1), (i, 2), \ldots, (i, N)$.
-----Output-----
For each test case, print a single line containing one integer ― the smallest number of operations required to sort the matrix.
-----Constraints-----
- $4 \le N \le 64$
- the sum of $N^2$ over all test files does not exceed $3 \cdot 10^5$
-----Subtasks-----
Subtask #1 (10 points):
- $T \le 50$
- $N = 4$
Subtask #2 (90 points): original constraints
-----Example Input-----
1
4
1 2 9 13
5 6 10 14
3 7 11 15
4 8 12 16
-----Example Output-----
2
-----Explanation-----
Example case 1: After the first operation, with $L = 2$, the resulting matrix is
1 5 9 13
2 6 10 14
3 7 11 15
4 8 12 16
After the second operation, with $L = 4$, the matrix becomes sorted
1 2 3 4
5 6 7 8
9 10 11 12
13 14 15 16 | # cook your dish here
import sys
t=int(input().strip())
for _ in range(t):
n=int(input().strip())
array=[]
for i in range(n):
lista=[]
lista[:]=map(int,input().strip().split())
array.append(lista)
j=n-1
i=0
counter=0
for _ in range(n):
if array[i][j]!=i+j+1:
counter+=1
if j>0:
j-=1
else:
i-=1
i, j= j, i
else:
if j>0:
j-=1
else:
i-=1
print(counter) | # cook your dish here
import sys
t=int(input().strip())
for _ in range(t):
n=int(input().strip())
array=[]
for i in range(n):
lista=[]
lista[:]=map(int,input().strip().split())
array.append(lista)
j=n-1
i=0
counter=0
for _ in range(n):
if array[i][j]!=i+j+1:
counter+=1
if j>0:
j-=1
else:
i-=1
i, j= j, i
else:
if j>0:
j-=1
else:
i-=1
print(counter) | train | APPS_structured |
As we all know, Max is the best video game player among her friends. Her friends were so jealous of hers, that they created an actual game just to prove that she's not the best at games. The game is played on a directed acyclic graph (a DAG) with n vertices and m edges. There's a character written on each edge, a lowercase English letter.
[Image]
Max and Lucas are playing the game. Max goes first, then Lucas, then Max again and so on. Each player has a marble, initially located at some vertex. Each player in his/her turn should move his/her marble along some edge (a player can move the marble from vertex v to vertex u if there's an outgoing edge from v to u). If the player moves his/her marble from vertex v to vertex u, the "character" of that round is the character written on the edge from v to u. There's one additional rule; the ASCII code of character of round i should be greater than or equal to the ASCII code of character of round i - 1 (for i > 1). The rounds are numbered for both players together, i. e. Max goes in odd numbers, Lucas goes in even numbers. The player that can't make a move loses the game. The marbles may be at the same vertex at the same time.
Since the game could take a while and Lucas and Max have to focus on finding Dart, they don't have time to play. So they asked you, if they both play optimally, who wins the game?
You have to determine the winner of the game for all initial positions of the marbles.
-----Input-----
The first line of input contains two integers n and m (2 ≤ n ≤ 100, $1 \leq m \leq \frac{n(n - 1)}{2}$).
The next m lines contain the edges. Each line contains two integers v, u and a lowercase English letter c, meaning there's an edge from v to u written c on it (1 ≤ v, u ≤ n, v ≠ u). There's at most one edge between any pair of vertices. It is guaranteed that the graph is acyclic.
-----Output-----
Print n lines, a string of length n in each one. The j-th character in i-th line should be 'A' if Max will win the game in case her marble is initially at vertex i and Lucas's marble is initially at vertex j, and 'B' otherwise.
-----Examples-----
Input
4 4
1 2 b
1 3 a
2 4 c
3 4 b
Output
BAAA
ABAA
BBBA
BBBB
Input
5 8
5 3 h
1 2 c
3 1 c
3 2 r
5 1 r
4 3 z
5 4 r
5 2 h
Output
BABBB
BBBBB
AABBB
AAABA
AAAAB
-----Note-----
Here's the graph in the first sample test case:
[Image]
Here's the graph in the second sample test case:
[Image] | # int(input())
# [int(i) for i in input().split()]
import sys
sys.setrecursionlimit(20000)
def go(v,w,last):
if game[v][w][last] >= 0: return(game[v][w][last])
flag = 0
move = 0
for p in edges_out[v]:
if p[1] >= last:
move = 1
if not go(w,p[0],p[1]):
flag = 1
break
if not move or not flag:
game[v][w][last] = 0
return(0)
else:
game[v][w][last] = 1
return(1)
n,m = [int(i) for i in input().split()]
edges_in = []
edges_out = []
for i in range(n):
edges_in.append([])
edges_out.append([])
for i in range(m):
s1,s2,s3 = input().split()
v = int(s1)-1
w = int(s2)-1
weight = ord(s3[0]) - ord('a') + 1
edges_out[v].append((w,weight))
edges_in[w].append((v,weight))
game = []
for i in range(n):
tmp1 = []
for j in range(n):
tmp2 = []
for c in range(27):
tmp2.append(-1)
tmp1.append(tmp2)
game.append(tmp1)
##for v in range(n):
## for w in range(n):
## for last in range(27):
## go(v,w,last)
for v in range(n):
s = ''
for w in range(n):
if go(v,w,0): s = s + 'A'
else: s = s + 'B'
print(s)
# Made By Mostafa_Khaled
| # int(input())
# [int(i) for i in input().split()]
import sys
sys.setrecursionlimit(20000)
def go(v,w,last):
if game[v][w][last] >= 0: return(game[v][w][last])
flag = 0
move = 0
for p in edges_out[v]:
if p[1] >= last:
move = 1
if not go(w,p[0],p[1]):
flag = 1
break
if not move or not flag:
game[v][w][last] = 0
return(0)
else:
game[v][w][last] = 1
return(1)
n,m = [int(i) for i in input().split()]
edges_in = []
edges_out = []
for i in range(n):
edges_in.append([])
edges_out.append([])
for i in range(m):
s1,s2,s3 = input().split()
v = int(s1)-1
w = int(s2)-1
weight = ord(s3[0]) - ord('a') + 1
edges_out[v].append((w,weight))
edges_in[w].append((v,weight))
game = []
for i in range(n):
tmp1 = []
for j in range(n):
tmp2 = []
for c in range(27):
tmp2.append(-1)
tmp1.append(tmp2)
game.append(tmp1)
##for v in range(n):
## for w in range(n):
## for last in range(27):
## go(v,w,last)
for v in range(n):
s = ''
for w in range(n):
if go(v,w,0): s = s + 'A'
else: s = s + 'B'
print(s)
# Made By Mostafa_Khaled
| train | APPS_structured |
Peter can see a clock in the mirror from the place he sits in the office.
When he saw the clock shows 12:22
1
2
3
4
5
6
7
8
9
10
11
12
He knows that the time is 11:38
1
2
3
4
5
6
7
8
9
10
11
12
in the same manner:
05:25 --> 06:35
01:50 --> 10:10
11:58 --> 12:02
12:01 --> 11:59
Please complete the function `WhatIsTheTime(timeInMirror)`, where `timeInMirror` is the mirrored time (what Peter sees) as string.
Return the _real_ time as a string.
Consider hours to be between 1 <= hour < 13.
So there is no 00:20, instead it is 12:20.
There is no 13:20, instead it is 01:20. | def what_is_the_time(time_in_mirror):
hours, minutes = list(map(int, time_in_mirror.split(':')))
if minutes == 0:
mirrored_hours = 12 - hours
mirrored_minutes = 0
else:
mirrored_hours = (11 - hours) % 12
mirrored_minutes = 60 - minutes
if mirrored_hours == 0:
mirrored_hours = 12
return "{:02d}:{:02d}".format(mirrored_hours, mirrored_minutes)
| def what_is_the_time(time_in_mirror):
hours, minutes = list(map(int, time_in_mirror.split(':')))
if minutes == 0:
mirrored_hours = 12 - hours
mirrored_minutes = 0
else:
mirrored_hours = (11 - hours) % 12
mirrored_minutes = 60 - minutes
if mirrored_hours == 0:
mirrored_hours = 12
return "{:02d}:{:02d}".format(mirrored_hours, mirrored_minutes)
| train | APPS_structured |
Integral numbers can be even or odd.
Even numbers satisfy `n = 2m` ( with `m` also integral ) and we will ( completely arbitrarily ) think of odd numbers as `n = 2m + 1`.
Now, some odd numbers can be more odd than others: when for some `n`, `m` is more odd than for another's. Recursively. :]
Even numbers are just not odd.
# Task
Given a finite list of integral ( not necessarily non-negative ) numbers, determine the number that is _odder than the rest_.
If there is no single such number, no number is odder than the rest; return `Nothing`, `null` or a similar empty value.
# Examples
```python
oddest([1,2]) => 1
oddest([1,3]) => 3
oddest([1,5]) => None
```
# Hint
Do you _really_ want one? Point or tap here. | def oddest(numbers):
most_odd = 0 # The current most odd number in the list
max_oddity = -1 # The current greatest oddity rate, starts at -1 so that even an even number can be the unique most odd
is_unique = True # If the current most odd number is really the most, so if there is no unique oddest number, it will return None
for num in numbers: # Loop through all the numbers
oddity = 0 # The oddity rate starts at 0
print(num)
insider = num # The coefficient of the number 2, so in 2n + 1, the insider is n
while insider % 2 == 1: # While that coefficient is odd
if insider == -1:
oddity = 1 + max([abs(n) for n in numbers]) # Since the oddity rate of a number is NEVER greater than the absolute value of the number, this garantees that the current number is the most odd one
break
else:
oddity += 1 # Add the oddity rate of the total number
insider = (insider-1)/2 # So if in 2n + 1, n is odd, represent it as 2(2m + 1) + 1, and set the value to m
if oddity > max_oddity: # If the current number's oddity rate is greater than the current max oddity,
is_unique = True # Set it to unique
max_oddity = oddity # Set the max oddity to the current oddity
most_odd = num # Set the most odd number to the current number
elif oddity == max_oddity:# Otherwise, if it's the same rate
is_unique = False # It's not unique
if is_unique and max_oddity >= 0: # If the current most odd number is REALLY the most odd number and the list isn't empty
return most_odd # Return it
return None # Otherwise, return None | def oddest(numbers):
most_odd = 0 # The current most odd number in the list
max_oddity = -1 # The current greatest oddity rate, starts at -1 so that even an even number can be the unique most odd
is_unique = True # If the current most odd number is really the most, so if there is no unique oddest number, it will return None
for num in numbers: # Loop through all the numbers
oddity = 0 # The oddity rate starts at 0
print(num)
insider = num # The coefficient of the number 2, so in 2n + 1, the insider is n
while insider % 2 == 1: # While that coefficient is odd
if insider == -1:
oddity = 1 + max([abs(n) for n in numbers]) # Since the oddity rate of a number is NEVER greater than the absolute value of the number, this garantees that the current number is the most odd one
break
else:
oddity += 1 # Add the oddity rate of the total number
insider = (insider-1)/2 # So if in 2n + 1, n is odd, represent it as 2(2m + 1) + 1, and set the value to m
if oddity > max_oddity: # If the current number's oddity rate is greater than the current max oddity,
is_unique = True # Set it to unique
max_oddity = oddity # Set the max oddity to the current oddity
most_odd = num # Set the most odd number to the current number
elif oddity == max_oddity:# Otherwise, if it's the same rate
is_unique = False # It's not unique
if is_unique and max_oddity >= 0: # If the current most odd number is REALLY the most odd number and the list isn't empty
return most_odd # Return it
return None # Otherwise, return None | train | APPS_structured |
# Task
The string is called `prime` if it cannot be constructed by concatenating some (more than one) equal strings together.
For example, "abac" is prime, but "xyxy" is not("xyxy"="xy"+"xy").
Given a string determine if it is prime or not.
# Input/Output
- `[input]` string `s`
string containing only lowercase English letters
- `[output]` a boolean value
`true` if the string is prime, `false` otherwise | def prime_string(s):
n=len(s)
return all(s!= s[:i]*(n//i) for i in range(1,n//2 +1))
| def prime_string(s):
n=len(s)
return all(s!= s[:i]*(n//i) for i in range(1,n//2 +1))
| train | APPS_structured |
Navnit is a college student and there are $N$ students in his college .Students are numbered from $1$ to $N$.
You are given $M$ facts that "Student $A_i$ and $B_i$".The same fact can be given multiple times .If $A_i$ is a friend of $B_i$ ,then $B_i$ is also a friend of $A_i$ . If $A_i$ is a friend of $B_i$ and $B_i$ is a friend of $C_i$ then $A_i$ is also a friend of $C_i$.
Find number of ways in which two students can be selected in such a way that they are not friends.
-----Input:-----
- First line will contain two integers $N$ and $M$.
- Then $M$ lines follow. Each line contains two integers $A_i$ and $B_i$ denoting the students who are friends.
-----Output:-----
For each testcase, output the number of ways in which two students can be selected in such a way that they are friends.
-----Constraints-----
- $2 \leq N \leq 200000$
- $0 \leq M \leq 200000$
- $1 \leq A_i,B_i \leq N$
-----Sample Input:-----
5 3
1 2
3 4
1 5
-----Sample Output:-----
6
-----EXPLANATION:-----
Groups of friend are $[1,2,5]$ and $[3,4]$.Hence the answer is 3 X 2 =6. | # cook your dish here
from collections import defaultdict
d=defaultdict(list)
def dfs(i):
p=0
nonlocal v
e=[i]
while(e!=[]):
p+=1
x=e.pop(0)
v[x]=1
for i in d[x]:
if v[i]==-1:
v[i]=1
e.append(i)
return p
n,m=list(map(int,input().split()))
for i in range(n+1):
d[i]=[]
for _ in range(m):
a,b=list(map(int,input().split()))
d[a].append(b)
d[b].append(a)
v=[]
for i in range(n+1):
v.append(-1)
c=0
p=[]
for i in range(1,n+1):
if v[i]==-1:
c+=1
p.append(dfs(i))
an=0
s=0
for i in range(c):
s+=p[i]
an+=p[i]*(n-s)
print(an)
| # cook your dish here
from collections import defaultdict
d=defaultdict(list)
def dfs(i):
p=0
nonlocal v
e=[i]
while(e!=[]):
p+=1
x=e.pop(0)
v[x]=1
for i in d[x]:
if v[i]==-1:
v[i]=1
e.append(i)
return p
n,m=list(map(int,input().split()))
for i in range(n+1):
d[i]=[]
for _ in range(m):
a,b=list(map(int,input().split()))
d[a].append(b)
d[b].append(a)
v=[]
for i in range(n+1):
v.append(-1)
c=0
p=[]
for i in range(1,n+1):
if v[i]==-1:
c+=1
p.append(dfs(i))
an=0
s=0
for i in range(c):
s+=p[i]
an+=p[i]*(n-s)
print(an)
| train | APPS_structured |
Consider the string `"adfa"` and the following rules:
```Pearl
a) each character MUST be changed either to the one before or the one after in alphabet.
b) "a" can only be changed to "b" and "z" to "y".
```
From our string, we get:
```Pearl
"adfa" -> ["begb","beeb","bcgb","bceb"]
Another example: "bd" -> ["ae","ac","ce","cc"]
--We see that in each example, one of the possibilities is a palindrome.
```
I was working on the code for this but I couldn't quite figure it out. So far I have:
```python
def solve(st):
return [all(ord(x) - ord(y) in ["FIX"] for x, y in zip(st, st[::-1]))][0]
```
I'm not sure what three numbers go into the array labelled `["FIX"]`. This is the only thing missing.
You will be given a lowercase string and your task is to return `True` if at least one of the possiblities is a palindrome or `False` otherwise. You can use your own code or fix mine.
More examples in test cases. Good luck! | def solve(st):
return all(True if ord(x) - ord(y) in [-2, 0, 2] else False for x, y in zip(st, st[::-1]))
| def solve(st):
return all(True if ord(x) - ord(y) in [-2, 0, 2] else False for x, y in zip(st, st[::-1]))
| train | APPS_structured |
Chef played an interesting game yesterday. This game is played with two variables $X$ and $Y$; initially, $X = Y = 0$. Chef may make an arbitrary number of moves (including zero). In each move, he must perform the following process:
- Choose any positive integer $P$ such that $P \cdot P > Y$.
- Change $X$ to $P$.
- Add $P \cdot P$ to $Y$.
Unfortunately, Chef has a bad memory and he has forgotten the moves he made. He only remembers the value of $X$ after the game finished; let's denote it by $X_f$. Can you tell him the maximum possible number of moves he could have made in the game?
-----Input-----
- The first line of the input contains a single integer $T$ denoting the number of test cases. The description of $T$ test cases follows.
- The first and only line of each test case contains a single integer $X_f$.
-----Output-----
For each test case, print a single line containing one integer — the maximum number of moves Chef could have made.
-----Constraints-----
- $1 \le T \le 10^5$
- $1 \le X_f \le 10^9$
-----Example Input-----
3
3
8
9
-----Example Output-----
3
5
6
-----Explanation-----
Example case 2: One possible sequence of values of $X$ is $0 \rightarrow 1 \rightarrow 2 \rightarrow 3 \rightarrow 5 \rightarrow 8$. | from math import ceil
for i in range(int(input())):
xf=int(input())
x=0
y=0
p=1
step=0
while(x<=xf):
x=p
y+=(p*p)
p=ceil(y**0.5)
step+=1
print(step-2) | from math import ceil
for i in range(int(input())):
xf=int(input())
x=0
y=0
p=1
step=0
while(x<=xf):
x=p
y+=(p*p)
p=ceil(y**0.5)
step+=1
print(step-2) | train | APPS_structured |
Write a function, `gooseFilter` / `goose-filter` / `goose_filter` /` GooseFilter`, that takes an array of strings as an argument and returns a filtered array containing the same elements but with the 'geese' removed.
The geese are any strings in the following array, which is pre-populated in your solution:
```python
geese = ["African", "Roman Tufted", "Toulouse", "Pilgrim", "Steinbacher"]
```
For example, if this array were passed as an argument:
```python
["Mallard", "Hook Bill", "African", "Crested", "Pilgrim", "Toulouse", "Blue Swedish"]
```
Your function would return the following array:
```python
["Mallard", "Hook Bill", "Crested", "Blue Swedish"]
```
The elements in the returned array should be in the same order as in the initial array passed to your function, albeit with the 'geese' removed. Note that all of the strings will be in the same case as those provided, and some elements may be repeated. | geese = ["African", "Roman Tufted", "Toulouse", "Pilgrim", "Steinbacher"]
def goose_filter(birds):
result = list(filter(lambda x:x not in geese,birds))
return result | geese = ["African", "Roman Tufted", "Toulouse", "Pilgrim", "Steinbacher"]
def goose_filter(birds):
result = list(filter(lambda x:x not in geese,birds))
return result | train | APPS_structured |
Complete the function that takes one argument, a list of words, and returns the length of the longest word in the list.
For example:
```python
['simple', 'is', 'better', 'than', 'complex'] ==> 7
```
Do not modify the input list. | def longest(words):
return len(max(words, key=len)) | def longest(words):
return len(max(words, key=len)) | train | APPS_structured |
Chef is a very experienced and well-known cook. He has participated in many cooking competitions in the past — so many that he does not even remember them all.
One of these competitions lasted for a certain number of days. The first day of the competition was day $S$ of the week (i.e. Monday, Tuesday etc.) and the last day was day $E$ of the week. Chef remembers that the duration of the competition (the number of days between the first and last day, inclusive) was between $L$ days and $R$ days inclusive. Is it possible to uniquely determine the exact duration of the competition? If so, what is this duration?
-----Input-----
- The first line of the input contains a single integer $T$ denoting the number of test cases. The description of $T$ test cases follows.
- The first and only line of each test case contains two space-separated strings $S$ and $E$, followed by a space and two space-separated integers $L$ and $R$.
-----Output-----
For each test case, print a single line containing:
- the string "impossible" if there is no duration consistent with all given information
- the string "many" if there is more than one possible duration
- one integer — the duration of the competition, if its duration is unique
-----Constraints-----
- $1 \le T \le 10,000$
- $1 \le L \le R \le 100$
- $S$ is one of the strings "saturday", "sunday", "monday", "tuesday", "wednesday", "thursday" or "friday"
- $E$ is one of the strings "saturday", "sunday", "monday", "tuesday", "wednesday", "thursday" or "friday"
-----Subtasks-----
Subtask #1 (100 points): original constraints
-----Example Input-----
3
saturday sunday 2 4
monday wednesday 1 20
saturday sunday 3 5
-----Example Output-----
2
many
impossible | # cook your dish here
d=["saturday","sunday","monday","tuesday","wednesday","thursday","friday"]
t=int(input())
for i in range(t):
s,e,l,r=map(str,input().split())
l,r=int(l),int(r)
v=(d.index(e)-d.index(s)+8)%7
c=r+1
for i in range(l,r+1):
if i%7==v:
c=i
break
if c>r:
print('impossible')
elif c+7<=r:
print('many')
else:
print(c) | # cook your dish here
d=["saturday","sunday","monday","tuesday","wednesday","thursday","friday"]
t=int(input())
for i in range(t):
s,e,l,r=map(str,input().split())
l,r=int(l),int(r)
v=(d.index(e)-d.index(s)+8)%7
c=r+1
for i in range(l,r+1):
if i%7==v:
c=i
break
if c>r:
print('impossible')
elif c+7<=r:
print('many')
else:
print(c) | train | APPS_structured |
The task is simply stated. Given an integer n (3 < n < 10^(9)), find the length of the smallest list of [*perfect squares*](https://en.wikipedia.org/wiki/Square_number) which add up to n. Come up with the best algorithm you can; you'll need it!
Examples:
sum_of_squares(17) = 2 17 = 16 + 1 (4 and 1 are perfect squares).
sum_of_squares(15) = 4 15 = 9 + 4 + 1 + 1. There is no way to represent 15 as the sum of three perfect squares.
sum_of_squares(16) = 1 16 itself is a perfect square.
Time constraints:
5 easy (sample) test cases: n < 20
5 harder test cases: 1000 < n < 15000
5 maximally hard test cases: 5 * 1e8 < n < 1e9
```if:java
300 random maximally hard test cases: 1e8 < n < 1e9
```
```if:c#
350 random maximally hard test cases: 1e8 < n < 1e9
```
```if:python
15 random maximally hard test cases: 1e8 < n < 1e9
```
```if:ruby
25 random maximally hard test cases: 1e8 < n < 1e9
```
```if:javascript
100 random maximally hard test cases: 1e8 < n < 1e9
```
```if:crystal
250 random maximally hard test cases: 1e8 < n < 1e9
```
```if:cpp
Random maximally hard test cases: 1e8 < n < 1e9
``` | from math import sqrt
def sum_of_squares(n):
n_sqrt = int(sqrt(n))
if n == n_sqrt * n_sqrt:
return 1
div, mod = divmod(n, 4)
while mod == 0:
div, mod = divmod(div, 4)
if (div * 4 + mod) % 8 == 7:
return 4
for i in range(1, n_sqrt + 1):
temp = n - i * i
temp_sqrt = int(sqrt(temp))
if temp == temp_sqrt * temp_sqrt:
return 2
return 3 | from math import sqrt
def sum_of_squares(n):
n_sqrt = int(sqrt(n))
if n == n_sqrt * n_sqrt:
return 1
div, mod = divmod(n, 4)
while mod == 0:
div, mod = divmod(div, 4)
if (div * 4 + mod) % 8 == 7:
return 4
for i in range(1, n_sqrt + 1):
temp = n - i * i
temp_sqrt = int(sqrt(temp))
if temp == temp_sqrt * temp_sqrt:
return 2
return 3 | train | APPS_structured |
You need to swap the head and the tail of the specified array:
the head (the first half) of array moves to the end, the tail (the second half) moves to the start.
The middle element (if it exists) leaves on the same position.
Return new array.
For example:
```
[ 1, 2, 3, 4, 5 ] => [ 4, 5, 3, 1, 2 ]
\----/ \----/
head tail
[ -1, 2 ] => [ 2, -1 ]
[ 1, 2, -3, 4, 5, 6, -7, 8 ] => [ 5, 6, -7, 8, 1, 2, -3, 4 ]
``` | def swap_head_tail(lst):
n, d = divmod(len(lst), 2)
return lst[n+d:] + [lst[n]] * d + lst[:n] | def swap_head_tail(lst):
n, d = divmod(len(lst), 2)
return lst[n+d:] + [lst[n]] * d + lst[:n] | train | APPS_structured |
# Task
You are given an array of integers `arr` that representing coordinates of obstacles situated on a straight line.
Assume that you are jumping from the point with coordinate 0 to the right. You are allowed only to make jumps of the same length represented by some integer.
Find the minimal length of the jump enough to avoid all the obstacles.
# Example
For `arr = [5, 3, 6, 7, 9]`, the output should be `4`.
Check out the image below for better understanding:
# Input/Output
- `[input]` integer array `arr`
Non-empty array of positive integers.
Constraints: `1 ≤ inputArray[i] ≤ 100.`
- `[output]` an integer
The desired length. | def avoid_obstacles(arr):
n=2
while 1:
if all([x%n for x in arr]): return n
n+=1 | def avoid_obstacles(arr):
n=2
while 1:
if all([x%n for x in arr]): return n
n+=1 | train | APPS_structured |
###Introduction
The [I Ching](https://en.wikipedia.org/wiki/I_Ching) (Yijing, or Book of Changes) is an ancient Chinese book of sixty-four hexagrams.
A hexagram is a figure composed of six stacked horizontal lines, where each line is either Yang (an unbroken line) or Yin (a broken line):
```
--------- ---- ---- ---------
---- ---- ---- ---- ---------
---- ---- ---- ---- ---------
--------- ---- ---- ---- ----
--------- --------- ---- ----
---- ---- ---- ---- ---------
```
The book is commonly used as an oracle. After asking it a question about one's present scenario,
each line is determined by random methods to be Yang or Yin. The resulting hexagram is then interpreted by the querent as a symbol of their current situation, and how it might unfold.
This kata will consult the I Ching using the three coin method.
###Instructions
A coin is flipped three times and lands heads
or tails. The three results are used to
determine a line as being either:
```
3 tails ----x---- Yin (Moving Line*)
2 tails 1 heads --------- Yang
1 tails 2 heads ---- ---- Yin
3 heads ----o---- Yang (Moving Line*)
*See bottom of description if curious.
```
This process is repeated six times to determine
each line of the hexagram. The results of these
operations are then stored in a 2D Array like so:
In each array the first index will always be the number of the line ('one' is the bottom line, and 'six' the top), and the other three values will be the results of the coin flips that belong to that line as heads ('h') and tails ('t').
Write a function that will take a 2D Array like the above as argument and return its hexagram as a string. Each line of the hexagram should begin on a new line.
should return:
```
---------
---------
----x----
----o----
---- ----
---- ----
```
You are welcome to consult your new oracle program with a question before pressing submit. You can compare your result [here](http://www.ichingfortune.com/hexagrams.php). The last test case is random and can be used for your query.
*[1] A Moving Line is a Yang line that will change
to Yin or vice versa. The hexagram made by the coin
throws represents the querent's current situation,
and the hexagram that results from changing its
moving lines represents their upcoming situation.* | ORDER = ('six', 'five', 'four', 'three', 'two', 'one')
YIN_YANG = {'hhh': '----o----', 'hht': '---- ----',
'htt': '---------', 'ttt': '----x----'}
def oracle(arr):
return '\n'.join(YIN_YANG[''.join(sorted(a[1:]))] for a in
sorted(arr, key=lambda b: ORDER.index(b[0])))
| ORDER = ('six', 'five', 'four', 'three', 'two', 'one')
YIN_YANG = {'hhh': '----o----', 'hht': '---- ----',
'htt': '---------', 'ttt': '----x----'}
def oracle(arr):
return '\n'.join(YIN_YANG[''.join(sorted(a[1:]))] for a in
sorted(arr, key=lambda b: ORDER.index(b[0])))
| train | APPS_structured |
# Background
I drink too much coffee. Eventually it will probably kill me.
*Or will it..?*
Anyway, there's no way to know.
*Or is there...?*
# The Discovery of the Formula
I proudly announce my discovery of a formula for measuring the life-span of coffee drinkers!
For
* ```h``` is a health number assigned to each person (8 digit date of birth YYYYMMDD)
* ```CAFE``` is a cup of *regular* coffee
* ```DECAF``` is a cup of *decaffeinated* coffee
To determine the life-time coffee limits:
* Drink cups of coffee (i.e. add to ```h```) until any part of the health number includes `DEAD`
* If the test subject can survive drinking five thousand cups wihout being ```DEAD``` then coffee has no ill effect on them
# Kata Task
Given the test subject's date of birth, return an array describing their life-time coffee limits
```[ regular limit , decaffeinated limit ]```
## Notes
* The limits are ```0``` if the subject is unaffected as described above
* At least 1 cup must be consumed (Just thinking about coffee cannot kill you!)
# Examples
* John was born 19/Jan/1950 so ```h=19500119```. His coffee limits are ```[2645, 1162]``` which is only about 1 cup per week. You better cut back John...How are you feeling? You don't look so well.
* Susan (11/Dec/1965) is unaffected by decaffeinated coffee, but regular coffee is very bad for her ```[111, 0]```. Just stick to the decaf Susan.
* Elizabeth (28/Nov/1964) is allergic to decaffeinated coffee. Dead after only 11 cups ```[0, 11]```. Read the label carefully Lizzy! Is it worth the risk?
* Peter (4/Sep/1965) can drink as much coffee as he likes ```[0, 0]```. You're a legend Peter!!
Hint: https://en.wikipedia.org/wiki/Hexadecimal
*Note: A life-span prediction formula this accurate has got to be worth a lot of money to somebody! I am willing to sell my copyright to the highest bidder. Contact me via the discourse section of this Kata.* | def decToHex(n):
hexChart = {0:'0',1:'1',2:'2',3:'3',4:'4',5:'5',6:'6',7:'7',8:'8',9:'9',10:'A',11:'B',12:'C',13:'D',14:'E',15:'F'}
hexValue=''
while n:
hexValue = hexChart[n%16] + hexValue
n //=16
return hexValue
def hexToDec(string):
hex = "0123456789ABCDEF"
total=0
for index, i in enumerate(string):
value = hex.index(i)
power = len(string) - (index+1)
total+= (value)*(16**power)
return total
def coffee_limits(year,month,day):
cafe = hexToDec('CAFE')
cafeCups=0
h = int(str(year) + str(month).zfill(2) + str(day).zfill(2))
for i in range(5000):
h += cafe
cafeCups+=1
if 'DEAD' in decToHex(h):
break
h = int(str(year) + str(month).zfill(2) + str(day).zfill(2))
print (h)
decaf = hexToDec('DECAF')
decafCups=0
for i in range(5000):
h+= decaf
decafCups+=1
if 'DEAD' in decToHex(h):
break
return [cafeCups%5000,decafCups%5000] | def decToHex(n):
hexChart = {0:'0',1:'1',2:'2',3:'3',4:'4',5:'5',6:'6',7:'7',8:'8',9:'9',10:'A',11:'B',12:'C',13:'D',14:'E',15:'F'}
hexValue=''
while n:
hexValue = hexChart[n%16] + hexValue
n //=16
return hexValue
def hexToDec(string):
hex = "0123456789ABCDEF"
total=0
for index, i in enumerate(string):
value = hex.index(i)
power = len(string) - (index+1)
total+= (value)*(16**power)
return total
def coffee_limits(year,month,day):
cafe = hexToDec('CAFE')
cafeCups=0
h = int(str(year) + str(month).zfill(2) + str(day).zfill(2))
for i in range(5000):
h += cafe
cafeCups+=1
if 'DEAD' in decToHex(h):
break
h = int(str(year) + str(month).zfill(2) + str(day).zfill(2))
print (h)
decaf = hexToDec('DECAF')
decafCups=0
for i in range(5000):
h+= decaf
decafCups+=1
if 'DEAD' in decToHex(h):
break
return [cafeCups%5000,decafCups%5000] | train | APPS_structured |
You have a string S consisting of N uppercase English letters. You are allowed to perform at most one operation of following kind: Choose any position in the string, remove the character at that position and insert it back to any other place in the string.
Find the lexicographically smallest string you can achieve.
-----Input-----
The first line of the input contains an integer T denoting the number of test cases. The description of T test cases follows.
The first line of each test case contains the single integer N denoting length of string S.
The second line contains the string S.
-----Output-----
For each test case, output a single line containing the answer to the corresponding test case.
-----Constraints-----
- 1 ≤ T ≤ 50
- 1 ≤ N ≤ 50
- S will consist of uppercase English letters.
-----Example-----
Input:
2
4
DCBA
7
XYZZYZZ
Output:
ADCB
XYYZZZZ
-----Explanation-----
Example case 1. The optimal solution here is to choose the last character and put it in the beginning of the string. So the answer will be ADCB
Example case 2. The optimal solution here is to choose the 5-th character (1-based index) and put it between the 2-nd and the 3-rd characters. So the answer will be XYYZZZZ | def modify(s,i,j):
k = s[i]
del(s[i])
s.insert(j,k)
return(str(s))
for _ in range(int(input())):
n = int(input())
s = list(input())
ans = str(s)
for i in range(n):
for j in range(n):
if i != j:
ans = min(ans,modify(s,i,j))
k = s[j]
del(s[j])
s.insert(i,k)
for i in ans:
if ord(i) >= 65 and ord(i) <=90:
print(i,end="")
print() | def modify(s,i,j):
k = s[i]
del(s[i])
s.insert(j,k)
return(str(s))
for _ in range(int(input())):
n = int(input())
s = list(input())
ans = str(s)
for i in range(n):
for j in range(n):
if i != j:
ans = min(ans,modify(s,i,j))
k = s[j]
del(s[j])
s.insert(i,k)
for i in ans:
if ord(i) >= 65 and ord(i) <=90:
print(i,end="")
print() | train | APPS_structured |
We have the first value of a certain sequence, we will name it ```initVal```.
We define pattern list, ```patternL```, an array that has the differences between contiguous terms of the sequence.
``` E.g: patternL = [k1, k2, k3, k4]```
The terms of the sequence will be such values that:
```python
term1 = initVal
term2 - term1 = k1
term3 - term2 = k2
term4 - term3 = k3
term5 - term4 = k4
term6 - term5 = k1
term7 - term6 = k2
term8 - term7 = k3
term9 - term8 = k4
.... - ..... = ...
.... - ..... = ...
```
So the values of the differences between contiguous terms are cyclical and are repeated as the differences values of the pattern list stablishes.
Let's see an example with numbers:
```python
initVal = 10
patternL = [2, 1, 3]
term1 = 10
term2 = 12
term3 = 13
term4 = 16
term5 = 18
term6 = 19
term7 = 22 # and so on...
```
We can easily obtain the next terms of the sequence following the values in the pattern list.
We see that the sixth term of the sequence, ```19```, has the sum of its digits ```10```.
Make a function ```sumDig_nthTerm()```, that receives three arguments in this order
```sumDig_nthTerm(initVal, patternL, nthTerm(ordinal number of the term in the sequence)) ```
This function will output the sum of the digits of the n-th term of the sequence.
Let's see some cases for this function:
```python
sumDig_nthTerm(10, [2, 1, 3], 6) -----> 10 # because the sixth term is 19 sum of Dig = 1 + 9 = 10. The sequence up to the sixth-Term is: 10, 12, 13, 16, 18, 19
sumDig_nthTerm(10, [1, 2, 3], 15) ----> 10 # 37 is the 15-th term, and 3 + 7 = 10
```
Enjoy it and happy coding!! | def sumDig_nthTerm(base, cycle, k):
loop, remaining = divmod(k - 1, len(cycle))
term = base + loop * sum(cycle) + sum(cycle[:remaining])
return sum(int(d) for d in str(term)) | def sumDig_nthTerm(base, cycle, k):
loop, remaining = divmod(k - 1, len(cycle))
term = base + loop * sum(cycle) + sum(cycle[:remaining])
return sum(int(d) for d in str(term)) | train | APPS_structured |
You are given two binary strings $S$ and $P$, each with length $N$. A binary string contains only characters '0' and '1'. For each valid $i$, let's denote the $i$-th character of $S$ by $S_i$.
You have to convert the string $S$ into $P$ using zero or more operations. In one operation, you should choose two indices $i$ and $j$ ($1 \leq i < j \leq N$) such that $S_i$ is '1' and $S_j$ is '0', and swap $S_i$ with $S_j$.
Determine if it is possible to convert $S$ into $P$ by performing some operations.
-----Input-----
- The first line of the input contains a single integer $T$ denoting the number of test cases. The description of $T$ test cases follows.
- The first line of each test case contains a single integer $N$.
- The second line contains a single string $S$.
- The third line contains a single string $P$.
-----Output-----
For each test case, print a single line containing the string "Yes" if it is possible to convert $S$ into $P$ or "No" otherwise (without quotes).
-----Constraints-----
- $1 \leq T \leq 10^5$
- $1 \leq N \leq 10^5$
- $S$ and $P$ contain only characters '0' and '1'
- the sum of $N$ over all test cases does not exceed $10^5$
-----Subtasks-----
Subtask #1 (20 points):
- $N \leq 14$
- the sum of $N$ over all test cases does not exceed $100$
Subtask #2 (30 points): the sum of $N$ over all test cases does not exceed $1,000$
Subtask #3 (50 points): original constraints
-----Example Input-----
3
2
00
00
3
101
010
4
0110
0011
-----Example Output-----
Yes
No
Yes
-----Explanation-----
Example case 1: The strings are already equal.
Example case 2: It can be showed that it is impossible to convert $S$ into $P$.
Example case 3: You can swap $S_2$ with $S_4$. The strings will then be equal. | # cook your dish here
t = int(input())
for _ in range(t):
n = int(input())
s = input()
p = input()
o=0
z=0
o2=0
z2=0
for i in s:
if(i=='1'):
o+=1
else:
z+=1
flag=0
for i in p:
if(i=='1'):
o2+=1
else:
z2+=1
spareone=0
if(o==o2 and z == z2):
for i in range(n):
if(s[i]!=p[i]):
if(p[i]=='0'):
spareone+=1
if(p[i]=='1'):
if(spareone==0):
flag=1
break
else:
spareone=spareone-1
if(flag==0):
print("Yes")
else:
print("No")
else:
print("No")
| # cook your dish here
t = int(input())
for _ in range(t):
n = int(input())
s = input()
p = input()
o=0
z=0
o2=0
z2=0
for i in s:
if(i=='1'):
o+=1
else:
z+=1
flag=0
for i in p:
if(i=='1'):
o2+=1
else:
z2+=1
spareone=0
if(o==o2 and z == z2):
for i in range(n):
if(s[i]!=p[i]):
if(p[i]=='0'):
spareone+=1
if(p[i]=='1'):
if(spareone==0):
flag=1
break
else:
spareone=spareone-1
if(flag==0):
print("Yes")
else:
print("No")
else:
print("No")
| train | APPS_structured |
Implement the [Polybius square cipher](http://en.wikipedia.org/wiki/Polybius_square).
Replace every letter with a two digit number. The first digit is the row number, and the second digit is the column number of following square. Letters `'I'` and `'J'` are both 24 in this cipher:
table#polybius-square {width: 100px;}
table#polybius-square td {background-color: #2f2f2f;}
table#polybius-square th {background-color: #3f3f3f;}
12345
1ABCDE
2FGHI/JK
3LMNOP
4QRSTU
5VWXYZ
Input will be valid (only spaces and uppercase letters from A to Z), so no need to validate them.
## Examples
```python
polybius('A') # "11"
polybius('IJ') # "2424"
polybius('CODEWARS') # "1334141552114243"
polybius('POLYBIUS SQUARE CIPHER') # "3534315412244543 434145114215 132435231542"
``` | def polybius(text):
numeric_key = {"a": "11", "b": "12", "c": "13", "d": "14", "e": "15",
"f": "21", "g": "22", "h": "23", "i": "24", "j": "24", "k": "25",
"l": "31", "m": "32", "n": "33", "o": "34", "p": "35",
"q": "41", "r": "42", "s": "43", "t": "44", "u": "45",
"v": "51", "w": "52", "x": "53", "y": "54", "z": "55",
" ": " "
}
numeric_string = ""
for character in text.lower():
numeric_string += numeric_key[character]
return numeric_string | def polybius(text):
numeric_key = {"a": "11", "b": "12", "c": "13", "d": "14", "e": "15",
"f": "21", "g": "22", "h": "23", "i": "24", "j": "24", "k": "25",
"l": "31", "m": "32", "n": "33", "o": "34", "p": "35",
"q": "41", "r": "42", "s": "43", "t": "44", "u": "45",
"v": "51", "w": "52", "x": "53", "y": "54", "z": "55",
" ": " "
}
numeric_string = ""
for character in text.lower():
numeric_string += numeric_key[character]
return numeric_string | train | APPS_structured |
You are given $n$ points on the plane. The polygon formed from all the $n$ points is strictly convex, that is, the polygon is convex, and there are no three collinear points (i.e. lying in the same straight line). The points are numbered from $1$ to $n$, in clockwise order.
We define the distance between two points $p_1 = (x_1, y_1)$ and $p_2 = (x_2, y_2)$ as their Manhattan distance: $$d(p_1, p_2) = |x_1 - x_2| + |y_1 - y_2|.$$
Furthermore, we define the perimeter of a polygon, as the sum of Manhattan distances between all adjacent pairs of points on it; if the points on the polygon are ordered as $p_1, p_2, \ldots, p_k$ $(k \geq 3)$, then the perimeter of the polygon is $d(p_1, p_2) + d(p_2, p_3) + \ldots + d(p_k, p_1)$.
For some parameter $k$, let's consider all the polygons that can be formed from the given set of points, having any $k$ vertices, such that the polygon is not self-intersecting. For each such polygon, let's consider its perimeter. Over all such perimeters, we define $f(k)$ to be the maximal perimeter.
Please note, when checking whether a polygon is self-intersecting, that the edges of a polygon are still drawn as straight lines. For instance, in the following pictures:
[Image]
In the middle polygon, the order of points ($p_1, p_3, p_2, p_4$) is not valid, since it is a self-intersecting polygon. The right polygon (whose edges resemble the Manhattan distance) has the same order and is not self-intersecting, but we consider edges as straight lines. The correct way to draw this polygon is ($p_1, p_2, p_3, p_4$), which is the left polygon.
Your task is to compute $f(3), f(4), \ldots, f(n)$. In other words, find the maximum possible perimeter for each possible number of points (i.e. $3$ to $n$).
-----Input-----
The first line contains a single integer $n$ ($3 \leq n \leq 3\cdot 10^5$) — the number of points.
Each of the next $n$ lines contains two integers $x_i$ and $y_i$ ($-10^8 \leq x_i, y_i \leq 10^8$) — the coordinates of point $p_i$.
The set of points is guaranteed to be convex, all points are distinct, the points are ordered in clockwise order, and there will be no three collinear points.
-----Output-----
For each $i$ ($3\leq i\leq n$), output $f(i)$.
-----Examples-----
Input
4
2 4
4 3
3 0
1 3
Output
12 14
Input
3
0 0
0 2
2 0
Output
8
-----Note-----
In the first example, for $f(3)$, we consider four possible polygons: ($p_1, p_2, p_3$), with perimeter $12$. ($p_1, p_2, p_4$), with perimeter $8$. ($p_1, p_3, p_4$), with perimeter $12$. ($p_2, p_3, p_4$), with perimeter $12$.
For $f(4)$, there is only one option, taking all the given points. Its perimeter $14$.
In the second example, there is only one possible polygon. Its perimeter is $8$. | import sys
input = sys.stdin.readline
n = int(input())
x = []
y = []
for i in range(n):
xi, yi = map(int, input().split())
x.append(xi)
y.append(yi)
min_x = min(x)
max_x = max(x)
min_y = min(y)
max_y = max(y)
answer = 0
for i in range(n):
dx = max(max_x - x[i], x[i] - min_x)
dy = max(max_y - y[i], y[i] - min_y)
answer = max(answer, dx + dy)
print(2*answer, end = ' ')
for i in range(4, n + 1):
print(2*(max_x - min_x + max_y - min_y), end = ' ') | import sys
input = sys.stdin.readline
n = int(input())
x = []
y = []
for i in range(n):
xi, yi = map(int, input().split())
x.append(xi)
y.append(yi)
min_x = min(x)
max_x = max(x)
min_y = min(y)
max_y = max(y)
answer = 0
for i in range(n):
dx = max(max_x - x[i], x[i] - min_x)
dy = max(max_y - y[i], y[i] - min_y)
answer = max(answer, dx + dy)
print(2*answer, end = ' ')
for i in range(4, n + 1):
print(2*(max_x - min_x + max_y - min_y), end = ' ') | train | APPS_structured |
Recently, Dima met with Sasha in a philatelic store, and since then they are collecting coins together. Their favorite occupation is to sort collections of coins. Sasha likes having things in order, that is why he wants his coins to be arranged in a row in such a way that firstly come coins out of circulation, and then come coins still in circulation.
For arranging coins Dima uses the following algorithm. One step of his algorithm looks like the following:
He looks through all the coins from left to right; If he sees that the i-th coin is still in circulation, and (i + 1)-th coin is already out of circulation, he exchanges these two coins and continues watching coins from (i + 1)-th.
Dima repeats the procedure above until it happens that no two coins were exchanged during this procedure. Dima calls hardness of ordering the number of steps required for him according to the algorithm above to sort the sequence, e.g. the number of times he looks through the coins from the very beginning. For example, for the ordered sequence hardness of ordering equals one.
Today Sasha invited Dima and proposed him a game. First he puts n coins in a row, all of them are out of circulation. Then Sasha chooses one of the coins out of circulation and replaces it with a coin in circulation for n times. During this process Sasha constantly asks Dima what is the hardness of ordering of the sequence.
The task is more complicated because Dima should not touch the coins and he should determine hardness of ordering in his mind. Help Dima with this task.
-----Input-----
The first line contains single integer n (1 ≤ n ≤ 300 000) — number of coins that Sasha puts behind Dima.
Second line contains n distinct integers p_1, p_2, ..., p_{n} (1 ≤ p_{i} ≤ n) — positions that Sasha puts coins in circulation to. At first Sasha replaces coin located at position p_1, then coin located at position p_2 and so on. Coins are numbered from left to right.
-----Output-----
Print n + 1 numbers a_0, a_1, ..., a_{n}, where a_0 is a hardness of ordering at the beginning, a_1 is a hardness of ordering after the first replacement and so on.
-----Examples-----
Input
4
1 3 4 2
Output
1 2 3 2 1
Input
8
6 8 3 4 7 2 1 5
Output
1 2 2 3 4 3 4 5 1
-----Note-----
Let's denote as O coin out of circulation, and as X — coin is circulation.
At the first sample, initially in row there are coins that are not in circulation, so Dima will look through them from left to right and won't make any exchanges.
After replacement of the first coin with a coin in circulation, Dima will exchange this coin with next three times and after that he will finally look through the coins and finish the process.
XOOO → OOOX
After replacement of the third coin, Dima's actions look this way:
XOXO → OXOX → OOXX
After replacement of the fourth coin, Dima's actions look this way:
XOXX → OXXX
Finally, after replacement of the second coin, row becomes consisting of coins that are in circulation and Dima will look through coins from left to right without any exchanges. | n = int(input())
p = [int(x) for x in input().split()]
mx = n
a = [0]*(n+1)
ans = [1]
for i in range(0, n):
t = p[i]
a[t] = 1
while a[mx]==1 and mx>=1: mx-=1
ans.append(i+1-(n-mx)+1)
print(' '.join(map(str, ans)))
# Made By Mostafa_Khaled
| n = int(input())
p = [int(x) for x in input().split()]
mx = n
a = [0]*(n+1)
ans = [1]
for i in range(0, n):
t = p[i]
a[t] = 1
while a[mx]==1 and mx>=1: mx-=1
ans.append(i+1-(n-mx)+1)
print(' '.join(map(str, ans)))
# Made By Mostafa_Khaled
| train | APPS_structured |
Chef Tobby is playing a rapid fire with Bhuvan. He gives Bhuvan a string S and each time, Bhuvan has to guess whether there exists 2 equal subsequences in the string or not.
Bhuvan got a perfect score in the game with Chef Tobby. However, Chef Tobby has now asked Bhuvan to write a program that will do this automatically given a string S. Bhuvan is an intelligent man but he does not know how to write a code. Can you help him?
Find two different subsequences such that they are equal in their value, more formally, find two sequences of indices (a1, a2, ..., ak-1, ak) and (b1, b2, ..., bk-1, bk) such that:
- 1≤ ai, bi ≤ |S|
- ai < ai+1 for all valid i
- bi < bi+1 for all valid i
- Sai = Sbi for all valid i
- there exist at least one i such that ai is not equal to bi
-----Input section-----
The first line contains T, the number of test cases.
Each of the next T lines contain one string S each.
Input will only consist of lowercase english characters
-----Output section-----
For each test case, output "yes" or "no" (without quotes) as the solution to the problem.
-----Input constraints-----
1 ≤ T ≤ 1000
1 ≤ length of S ≤ 100
-----Sample Input-----
4
likecs
venivedivici
bhuvan
codechef
-----Sample Output-----
no
yes
no
yes
-----Explanation-----
In test case 2, one of the possible equal subsequence is "vi" and "vi". (one at position {0, 3} and other at {4, 7}, assuming 0-based indexing).
In test case 4, one of the possible equal subsequence is "ce" and "ce". (one at position {0, 3} and other at {4, 6}, assuming 0-based indexing). | for _ in range(int(input())):
S = input()
for i in list(set(S)):
if S.count(i) > 1:
print('yes')
break
else:
print('no') | for _ in range(int(input())):
S = input()
for i in list(set(S)):
if S.count(i) > 1:
print('yes')
break
else:
print('no') | train | APPS_structured |
As we all know caterpillars love to eat leaves. Usually, a caterpillar sits on leaf, eats as much of it as it can (or wants), then stretches out to its full length to reach a new leaf with its front end, and finally "hops" to it by contracting its back end to that leaf.
We have with us a very long, straight branch of a tree with leaves distributed uniformly along its length, and a set of caterpillars sitting on the first leaf. (Well, our leaves are big enough to accommodate upto $20$ caterpillars!). As time progresses our caterpillars eat and hop repeatedly, thereby damaging many leaves. Not all caterpillars are of the same length, so different caterpillars may eat different sets of leaves. We would like to find out the number of leaves that will be undamaged at the end of this eating spree. We assume that adjacent leaves are a unit distance apart and the length of the caterpillars is also given in the same unit.
For example suppose our branch had $20$ leaves (placed $1$ unit apart) and $3$ caterpillars of length $3, 2$ and $5$ units respectively. Then, first caterpillar would first eat leaf $1$, then hop to leaf $4$ and eat it and then hop to leaf $7$ and eat it and so on. So the first caterpillar would end up eating the leaves at positions $1,4,7,10,13,16$ and $19$. The second caterpillar would eat the leaves at positions $1,3,5,7,9,11,13,15,17$ and $19$. The third caterpillar would eat the leaves at positions $1,6,11$ and $16$. Thus we would have undamaged leaves at positions $2,8,12,14,18$ and $20$. So the answer to this example is $6$.
-----Input:-----
The first line of the input contains two integers $N$ and $K$, where $N$ is the number of leaves and $K$ is the number of caterpillars. Lines $2,3,...,K+1$ describe the lengths of the $K$ caterpillars. Line $i+1$ ($1 \leq i \leq K$) contains a single integer representing the length of the $i^{th}$ caterpillar.
-----Output:-----
A line containing a single integer, which is the number of leaves left on the branch after all the caterpillars have finished their eating spree.
-----Constraints:-----
- $1 \leq N \leq 1000000000$.
- $1 \leq K \leq 20$.
- The length of the caterpillars lie between $1$ and $N$.
- $50 \%$ of test cases will also satisfy $1 \leq N \leq 10000000$ and $1 \leq K \leq 16$.
-----Sample Input:-----
20 3
3
2
5
-----Sample Output:-----
6
-----Hint:-----
You may use $64$-bit integers (long long in C/C++) to avoid errors while multiplying large integers. The maximum value you can store in a $32$-bit integer is $2^{31}-1$, which is approximately $2 \cdot 10^9$. $64$-bit integers can store values greater than $10^{18}$. | from math import gcd
n, k = list(map(int, input().split()))
a = []
for i in range(k):
try:
a += list(map(int, input().split()))
except:
pass
ans = n
for i in range(1, 2**k):
b = bin(i)[2:].rjust(k, "0")
c = []
for j in range(k):
if(b[j] == '1'):
c.append(a[j])
lcm = c[0]
for j in c[1:]:
lcm *= j // gcd(lcm, j)
temp = ((n - 1) // lcm) + 1
if(b.count('1')&1):
ans -= temp
else:
ans += temp
print(ans)
| from math import gcd
n, k = list(map(int, input().split()))
a = []
for i in range(k):
try:
a += list(map(int, input().split()))
except:
pass
ans = n
for i in range(1, 2**k):
b = bin(i)[2:].rjust(k, "0")
c = []
for j in range(k):
if(b[j] == '1'):
c.append(a[j])
lcm = c[0]
for j in c[1:]:
lcm *= j // gcd(lcm, j)
temp = ((n - 1) // lcm) + 1
if(b.count('1')&1):
ans -= temp
else:
ans += temp
print(ans)
| train | APPS_structured |
Pete and his mate Phil are out in the countryside shooting clay pigeons with a shotgun - amazing fun.
They decide to have a competition. 3 rounds, 2 shots each. Winner is the one with the most hits.
Some of the clays have something attached to create lots of smoke when hit, guarenteed by the packaging to generate 'real excitement!' (genuinely this happened). None of the explosive things actually worked, but for this kata lets say they did.
For each round you will receive the following format:
[{P1:'XX', P2:'XO'}, true]
That is an array containing an object and a boolean. Pl represents Pete, P2 represents Phil. X represents a hit and O represents a miss. If the boolean is true, any hit is worth 2. If it is false, any hit is worth 1.
Find out who won. If it's Pete, return 'Pete Wins!'. If it is Phil, return 'Phil Wins!'. If the scores are equal, return 'Draw!'.
Note that as there are three rounds, the actual input (x) will look something like this:
[[{P1:'XX', P2:'XO'}, true], [{P1:'OX', P2:'OO'}, false], [{P1:'XX', P2:'OX'}, true]] | def shoot(results):
pete, phil = [sum(d[p].count('X') * (1+b) for d, b in results) for p in ['P1', 'P2']]
return 'Pete Wins!' if pete > phil else 'Phil Wins!' if phil > pete else 'Draw!' | def shoot(results):
pete, phil = [sum(d[p].count('X') * (1+b) for d, b in results) for p in ['P1', 'P2']]
return 'Pete Wins!' if pete > phil else 'Phil Wins!' if phil > pete else 'Draw!' | train | APPS_structured |
Given two sequences pushed and popped with distinct values, return true if and only if this could have been the result of a sequence of push and pop operations on an initially empty stack.
Example 1:
Input: pushed = [1,2,3,4,5], popped = [4,5,3,2,1]
Output: true
Explanation: We might do the following sequence:
push(1), push(2), push(3), push(4), pop() -> 4,
push(5), pop() -> 5, pop() -> 3, pop() -> 2, pop() -> 1
Example 2:
Input: pushed = [1,2,3,4,5], popped = [4,3,5,1,2]
Output: false
Explanation: 1 cannot be popped before 2.
Constraints:
0 <= pushed.length == popped.length <= 1000
0 <= pushed[i], popped[i] < 1000
pushed is a permutation of popped.
pushed and popped have distinct values. | class Solution:
def validateStackSequences(self, pushed: List[int], popped: List[int]) -> bool:
pushed.reverse()
popped.reverse()
stack = []
while pushed:
value = pushed.pop()
stack.append(value)
while stack and popped and stack[-1] == popped[-1]:
popped.pop()
stack.pop()
if not pushed and not popped:
return True
return False
| class Solution:
def validateStackSequences(self, pushed: List[int], popped: List[int]) -> bool:
pushed.reverse()
popped.reverse()
stack = []
while pushed:
value = pushed.pop()
stack.append(value)
while stack and popped and stack[-1] == popped[-1]:
popped.pop()
stack.pop()
if not pushed and not popped:
return True
return False
| train | APPS_structured |
-----Problem Statement-----
A classroom has several students, half of whom are boys and half of whom are girls. You need to arrange all of them in a line for the morning assembly such that the following conditions are satisfied:
- The students must be in order of non-decreasing height.
- Two boys or two girls must not be adjacent to each other.
You have been given the heights of the boys in the array $b$ and the heights of the girls in the array $g$. Find out whether you can arrange them in an order which satisfies the given conditions. Print "YES" if it is possible, or "NO" if it is not.
For example, let's say there are $n = 3$ boys and $n = 3$ girls, where the boys' heights are $b = [5, 3, 8]$ and the girls' heights are $g = [2, 4, 6]$. These students can be arranged in the order $[g_0, b_1, g_1, b_0, g_2, b_2]$, which is $[2, 3, 4, 5, 6, 8]$. Because this is in order of non-decreasing height, and no two boys or two girls are adjacent to each other, this satisfies the conditions. Therefore, the answer is "YES".
-----Input-----
- The first line contains an integer, $t$, denoting the number of test cases.
- The first line of each test case contains an integer, $n$, denoting the number of boys and girls in the classroom.
- The second line of each test case contains $n$ space separated integers, $b_1,b_2, ... b_n$, denoting the heights of the boys.
- The second line of each test case contains $n$ space separated integers, $g_1,g_2,... g_n$, denoting the heights of the girls.
-----Output-----
Print exactly $t$ lines. In the $i^{th}$ of them, print a single line containing "$YES$" without quotes if it is possible to arrange the students in the $i^{th}$ test case, or "$NO$" without quotes if it is not.
-----Constraints-----
- $1 \leq t \leq 10$
- $1 \leq n \leq 100$
- $1 \leq b_i, g_i \leq 100$
-----Sample Input-----
1
2
1 3
2 4
-----Sample Output-----
YES
-----EXPLANATION-----
The following arrangement would satisfy the given conditions: $[b_1, g_1, b_2, g_2]$. This is because the boys and girls and separated, and the height is in non-decreasing order. | import re
for _ in range(int(input())):
n = int(input())
b = list(map(int,input().split()))
g = list(map(int,input().split()))
a = sorted(b)
b = sorted(g)
i,j = 0,0
x = ""
cutt = 2
while i<n and j<n:
if a[i]<b[j]:
x+="b"
i+=1
elif a[i]==b[j]:
if len(x)==0:
x+="b"
cutt=1
i+=1
elif x[-1]=="b":
x+="g"
j+=1
else:
x+="b"
i+=1
else:
x+="g"
j+=1
while i<n:
x+="b"
i+=1
while j<n:
x+="g"
j+=1
w = re.findall(r"[b]{2}",x)
t = re.findall(r"[g]{2}",x)
if (w or t) and cutt ==1:
i=0
n=len(a)
j=0
x=""
while i<n and j<n:
if a[i]<b[j]:
x+="b"
i+=1
elif a[i]==b[j]:
if len(x)==0:
x+="g"
j+=1
elif x[-1]=="b":
x+="g"
j+=1
else:
x+="b"
i+=1
else:
x+="g"
j+=1
while i<n:
x+="b"
i+=1
while j<n:
x+="g"
j+=1
w = re.findall(r"[b]{2}",x)
t = re.findall(r"[g]{2}",x)
if w or t:
print("NO")
else:
print('YES')
elif w or t:
print("NO")
else:
print("YES") | import re
for _ in range(int(input())):
n = int(input())
b = list(map(int,input().split()))
g = list(map(int,input().split()))
a = sorted(b)
b = sorted(g)
i,j = 0,0
x = ""
cutt = 2
while i<n and j<n:
if a[i]<b[j]:
x+="b"
i+=1
elif a[i]==b[j]:
if len(x)==0:
x+="b"
cutt=1
i+=1
elif x[-1]=="b":
x+="g"
j+=1
else:
x+="b"
i+=1
else:
x+="g"
j+=1
while i<n:
x+="b"
i+=1
while j<n:
x+="g"
j+=1
w = re.findall(r"[b]{2}",x)
t = re.findall(r"[g]{2}",x)
if (w or t) and cutt ==1:
i=0
n=len(a)
j=0
x=""
while i<n and j<n:
if a[i]<b[j]:
x+="b"
i+=1
elif a[i]==b[j]:
if len(x)==0:
x+="g"
j+=1
elif x[-1]=="b":
x+="g"
j+=1
else:
x+="b"
i+=1
else:
x+="g"
j+=1
while i<n:
x+="b"
i+=1
while j<n:
x+="g"
j+=1
w = re.findall(r"[b]{2}",x)
t = re.findall(r"[g]{2}",x)
if w or t:
print("NO")
else:
print('YES')
elif w or t:
print("NO")
else:
print("YES") | train | APPS_structured |
# Task
In the Land Of Chess, bishops don't really like each other. In fact, when two bishops happen to stand on the same diagonal, they immediately rush towards the opposite ends of that same diagonal.
Given the initial positions (in chess notation) of two bishops, `bishop1` and `bishop2`, calculate their future positions. Keep in mind that bishops won't move unless they see each other along the same diagonal.
# Example
For `bishop1 = "d7" and bishop2 = "f5"`, the output should be `["c8", "h3"]`.
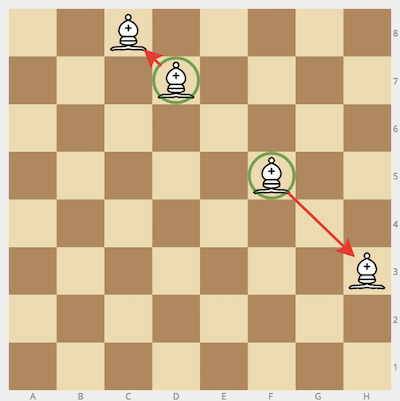
For `bishop1 = "d8" and bishop2 = "b5"`, the output should be `["b5", "d8"]`.
The bishops don't belong to the same diagonal, so they don't move.
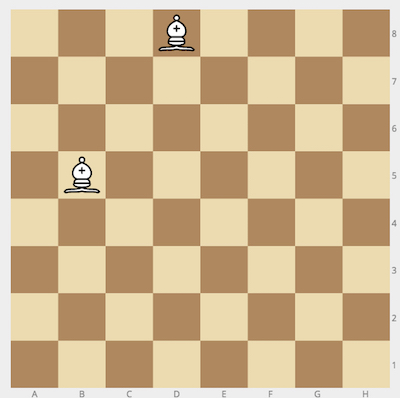
# Input/Output
- `[input]` string `bishop1`
Coordinates of the first bishop in chess notation.
- `[input]` string `bishop2`
Coordinates of the second bishop in the same notation.
- `[output]` a string array
Coordinates of the bishops in lexicographical order after they check the diagonals they stand on. | def bishop_diagonal(bishop1, bishop2):
rank, file = ord(bishop1[0]) - ord(bishop2[0]), int(bishop1[1]) - int(bishop2[1])
if abs(rank) == abs(file):
ranksign, filesign = -1 if rank < 0 else 1, -1 if file < 0 else 1
while bishop1[0] not in "ah" and bishop1[1] not in "18":
bishop1 = chr(ord(bishop1[0]) + ranksign) + str(int(bishop1[1]) + filesign)
while bishop2[0] not in "ah" and bishop2[1] not in "18":
bishop2 = chr(ord(bishop2[0]) - ranksign) + str(int(bishop2[1]) - filesign)
return sorted([bishop1, bishop2]) | def bishop_diagonal(bishop1, bishop2):
rank, file = ord(bishop1[0]) - ord(bishop2[0]), int(bishop1[1]) - int(bishop2[1])
if abs(rank) == abs(file):
ranksign, filesign = -1 if rank < 0 else 1, -1 if file < 0 else 1
while bishop1[0] not in "ah" and bishop1[1] not in "18":
bishop1 = chr(ord(bishop1[0]) + ranksign) + str(int(bishop1[1]) + filesign)
while bishop2[0] not in "ah" and bishop2[1] not in "18":
bishop2 = chr(ord(bishop2[0]) - ranksign) + str(int(bishop2[1]) - filesign)
return sorted([bishop1, bishop2]) | train | APPS_structured |
There are two types of soup: type A and type B. Initially we have N ml of each type of soup. There are four kinds of operations:
Serve 100 ml of soup A and 0 ml of soup B
Serve 75 ml of soup A and 25 ml of soup B
Serve 50 ml of soup A and 50 ml of soup B
Serve 25 ml of soup A and 75 ml of soup B
When we serve some soup, we give it to someone and we no longer have it. Each turn, we will choose from the four operations with equal probability 0.25. If the remaining volume of soup is not enough to complete the operation, we will serve as much as we can. We stop once we no longer have some quantity of both types of soup.
Note that we do not have the operation where all 100 ml's of soup B are used first.
Return the probability that soup A will be empty first, plus half the probability that A and B become empty at the same time.
Example:
Input: N = 50
Output: 0.625
Explanation:
If we choose the first two operations, A will become empty first. For the third operation, A and B will become empty at the same time. For the fourth operation, B will become empty first. So the total probability of A becoming empty first plus half the probability that A and B become empty at the same time, is 0.25 * (1 + 1 + 0.5 + 0) = 0.625.
Notes:
0 <= N <= 10^9.
Answers within 10^-6 of the true value will be accepted as correct. | class Solution:
def soupServings(self, N: int) -> float:
compressed_N = N // 25
N = compressed_N + (1 if (N-compressed_N*25)>0 else 0)
mem = {}
def dp(a, b):
if (a, b) in mem:
return mem[(a,b)]
else:
if a <= 0 or b <= 0:
result = 0.5 if a <=0 and b <= 0 else 1.0 if a <= 0 else 0.0
else:
result = 0.25 * (dp(a-4, b) + dp(a-3, b-1) + dp(a-2, b-2) + dp(a-1, b-3))
mem[(a,b)] = result
return result
if N <= 200:
return dp(N, N)
else:
for i in range(200, N+1):
current_result = dp(i, i)
if 1 - current_result < 1e-6:
return 1.0
return current_result
| class Solution:
def soupServings(self, N: int) -> float:
compressed_N = N // 25
N = compressed_N + (1 if (N-compressed_N*25)>0 else 0)
mem = {}
def dp(a, b):
if (a, b) in mem:
return mem[(a,b)]
else:
if a <= 0 or b <= 0:
result = 0.5 if a <=0 and b <= 0 else 1.0 if a <= 0 else 0.0
else:
result = 0.25 * (dp(a-4, b) + dp(a-3, b-1) + dp(a-2, b-2) + dp(a-1, b-3))
mem[(a,b)] = result
return result
if N <= 200:
return dp(N, N)
else:
for i in range(200, N+1):
current_result = dp(i, i)
if 1 - current_result < 1e-6:
return 1.0
return current_result
| train | APPS_structured |
Implement `String#digit?` (in Java `StringUtils.isDigit(String)`), which should return `true` if given object is a digit (0-9), `false` otherwise. | def is_digit(n):
#your code here: shortest variation
return n in set('0123456789') | def is_digit(n):
#your code here: shortest variation
return n in set('0123456789') | train | APPS_structured |
Given an array of unique integers salary where salary[i] is the salary of the employee i.
Return the average salary of employees excluding the minimum and maximum salary.
Example 1:
Input: salary = [4000,3000,1000,2000]
Output: 2500.00000
Explanation: Minimum salary and maximum salary are 1000 and 4000 respectively.
Average salary excluding minimum and maximum salary is (2000+3000)/2= 2500
Example 2:
Input: salary = [1000,2000,3000]
Output: 2000.00000
Explanation: Minimum salary and maximum salary are 1000 and 3000 respectively.
Average salary excluding minimum and maximum salary is (2000)/1= 2000
Example 3:
Input: salary = [6000,5000,4000,3000,2000,1000]
Output: 3500.00000
Example 4:
Input: salary = [8000,9000,2000,3000,6000,1000]
Output: 4750.00000
Constraints:
3 <= salary.length <= 100
10^3 <= salary[i] <= 10^6
salary[i] is unique.
Answers within 10^-5 of the actual value will be accepted as correct. | class Solution:
def average(self, salary: List[int]) -> float:
salary.sort()
del salary[0]
del salary[-1]
return sum(salary)/len(salary) | class Solution:
def average(self, salary: List[int]) -> float:
salary.sort()
del salary[0]
del salary[-1]
return sum(salary)/len(salary) | train | APPS_structured |
Simple enough this one - you will be given an array. The values in the array will either be numbers or strings, or a mix of both. You will not get an empty array, nor a sparse one.
Your job is to return a single array that has first the numbers sorted in ascending order, followed by the strings sorted in alphabetic order. The values must maintain their original type.
Note that numbers written as strings are strings and must be sorted with the other strings. | def db_sort(arr):
num = []
alpha = []
for i in arr:
if isinstance(i, int):
num.append(int(i))
else:
alpha.append(i)
num.sort()
alpha.sort()
return num + alpha | def db_sort(arr):
num = []
alpha = []
for i in arr:
if isinstance(i, int):
num.append(int(i))
else:
alpha.append(i)
num.sort()
alpha.sort()
return num + alpha | train | APPS_structured |
Meanwhile, Candace and Stacy are busy planning to attend the concert of the famous Love Händel. Jeremy will also be attending the event. Therefore, Candace plans to offer the best possible gift to Jeremy.
Candace has $N$ strings, each of length $M$. Each character of each string can either be a lowercase English letter or the character '#'. She denotes the substring of $i$-$th$ string starting from the $l$-$th$ character and ending with the $r$-$th$ character as $S_{i}[l, r]$. She has to choose a list $a$ of $N - 1$ numbers such that
- $1 \leq a_1 \leq a_2 \leq a_3 \leq ... \leq a_{N-1} \leq M$.
- The final gift string is obtained by appending the substrings $S_{1}[1, a_{1}]$, $S_{2}[a_{1}, a_{2}]$, $S_{3}[a_{2}, a_{3}]$, .., $S_{N-1}[a_{N-2}, a_{N-1}]$, $S_{N}[a_{N-1}, M]$.
- Candace considers the gift to be the most beautiful one if the final gift string is the lexicographically smallest possible string and it doesn't contain the '#' character.
Let $P$ = $S_{1}[1, a_{1}]$ + $S_{2}[a_{1}, a_{2}]$ + $S_{3}[a_{2}, a_{3}]$ + .., + $S_{N-1}[a_{N-2}, a_{N-1}]$ + $S_{N}[a_{N-1}, M]$, then $P$ should be the lexicographically smallest string possible and it should not contain '#' character, where '+' denotes concatenation of two substrings.
Help Candace find the best possible gift string quickly as she does not want Jeremy to wait for her. Also, it is guaranteed that at least one such valid string exists.
-----Input-----
- The first line contains a single integer $T$ denoting the number of testcases.
- The first line of each test case contains $2$ space separated integers denoting the values of $N$ and $M$ respectively.
- $N$ lines follow. Each line contains a string $S_i$ of length $M$ as described above.
-----Output-----
For every test case, print a line containing lexicographically lowest possible gift string.
-----Constraints-----
- $1 \leq T \leq 10^4$
- $1 \leq N, M \leq 10^6$
- $|S_i| = M$
- All $S_i$ will contain either a lower English letter ('a' to 'z' ) or the character '#' (ASCII $35$).
- Sum of $N * M$ over all test cases for a particular test file does not exceed $10^7$.
- It is guaranteed that a valid solution will always exist.
-----Sample Input-----
2
3 3
xab
a#z
caa
5 4
pyqs
vcot
qbiu
lihj
uvmz
-----Sample Output-----
xabza
pvcbihjz
-----Explanation-----
For test $1$:
The array $a$ chosen is: $[3, 3]$
The resulting string $P$ is formed by = $S_{1}[1, 3]$ + $S_{2}[3, 3]$ + $S_{3}[3, 3]$ = xabza
For test $2$:
The array $a$ chosen is: $[1, 2, 3, 4]$
The resulting string $P$ is formed by = $S_{1}[1, 1]$ + $S_{2}[1, 2]$ + $S_{3}[2, 3]$ + $S_{4}[3, 4]$ + $S_{5}[4, 4]$ = pvcbihjz | # import all important libraries and inbuilt functions
from fractions import Fraction
import numpy as np
import sys,bisect,copyreg,copy,statistics,os
from math import *
from collections import Counter,defaultdict,deque,OrderedDict
from itertools import combinations,permutations,accumulate
from numpy.linalg import matrix_power as mp
from bisect import bisect_left,bisect_right,bisect,insort,insort_left,insort_right
from statistics import mode
from functools import reduce,cmp_to_key
from io import BytesIO, IOBase
from scipy.spatial import ConvexHull
from heapq import *
from decimal import *
from queue import Queue,PriorityQueue
from re import sub,subn
# end of library import
# map system version faults
if sys.version_info[0] < 3:
from builtins import xrange as range
from future_builtins import ascii, filter, hex, map, oct, zip
# template of many functions used in competitive programming can add more later
# based on need we will use this commonly.
# bfs in a graph
def bfs(adj,v): # a schema of bfs
visited=[False]*(v+1);q=deque()
while q:pass
# definition of vertex of a graph
def graph(vertex): return [[] for i in range(vertex+1)]
def powermodulo(x, y, p) :
res = 1;x = x % p
if (x == 0) : return 0
while (y > 0) :
if ((y & 1) == 1) : res = (res * x) % p
y = y >> 1
x = (x * x) % p
return res
def lcm(a,b): return (a*b)//gcd(a,b)
# most common list in a array of lists
def most_frequent(List):return Counter(List).most_common(1)[0][0]
# element with highest frequency
def most_common(List):return(mode(List))
#In number theory, the Chinese remainder theorem states that
#if one knows the remainders of the Euclidean division of an integer n by
#several integers, then one can determine uniquely the remainder of the
#division of n by the product of these integers, under the condition
#that the divisors are pairwise coprime.
def chinese_remainder(a, p):
prod = reduce(op.mul, p, 1);x = [prod // pi for pi in p]
return sum(a[i] * powermodulo(x[i], p[i] - 2, p[i]) * x[i] for i in range(len(a))) % prod
# make a matrix
def createMatrix(rowCount, colCount, dataList):
mat = []
for i in range (rowCount):
rowList = []
for j in range (colCount):
if dataList[j] not in mat:rowList.append(dataList[j])
mat.append(rowList)
return mat
# input for a binary tree
def readTree():
v=int(inp());adj=[set() for i in range(v+1)]
for i in range(v-1):u1,u2=In(); adj[u1].add(u2);adj[u2].add(u1)
return adj,v
# sieve of prime numbers
def sieve():
li=[True]*1000001;li[0],li[1]=False,False;prime=[]
for i in range(2,len(li),1):
if li[i]==True:
for j in range(i*i,len(li),i):li[j]=False
for i in range(1000001):
if li[i]==True:prime.append(i)
return prime
#count setbits of a number.
def setBit(n):
count=0
while n!=0:n=n&(n-1);count+=1
return count
# sum of digits of a number
def digitsSum(n):
if n == 0:return 0
r = 0
while n > 0:r += n % 10;n //= 10
return r
# ncr efficiently
def ncr(n, r):
r = min(r, n - r);numer = reduce(op.mul, list(range(n, n - r, -1)), 1);denom = reduce(op.mul, list(range(1, r + 1)), 1)
return numer // denom # or / in Python 2
#factors of a number
def factors(n):return list(set(reduce(list.__add__, ([i, n // i] for i in range(1, int(n**0.5) + 1) if n % i == 0))))
#prime fators of a number
def prime_factors(n):
i = 2;factors = []
while i * i <= n:
if n % i:i += 1
else:n //= i;factors.append(i)
if n > 1:factors.append(n)
return len(set(factors))
def prefixSum(arr):
for i in range(1, len(arr)):arr[i] = arr[i] + arr[i-1]
return arr
def binomial_coefficient(n, k):
if 0 <= k <= n:
ntok = 1;ktok = 1
for t in range(1, min(k, n - k) + 1):ntok *= n;ktok *= t;n -= 1
return ntok // ktok
else:return 0
def powerOfK(k, max):
if k == 1:return [1]
if k == -1:return [-1, 1]
result = [];n = 1
while n <= max:result.append(n);n *= k
return result
# maximum subarray sum use kadane's algorithm
def kadane(a,size):
max_so_far = 0;max_ending_here = 0
for i in range(0, size):
max_ending_here = max_ending_here + a[i]
if (max_so_far < max_ending_here):max_so_far = max_ending_here
if max_ending_here < 0:max_ending_here = 0
return max_so_far
def divisors(n):
result = []
for i in range(1,ceil(sqrt(n))+1):
if n%i == 0:
if n/i == i:result.append(i)
else:result.append(i);result.append(n/i)
return result
def sumtilln(n): return ((n*(n+1))//2)
def isPrime(n) :
if (n <= 1) :return False
if (n <= 3) :return True
if (n % 2 == 0 or n % 3 == 0) :return False
for i in range(5,ceil(sqrt(n))+1,6):
if (n % i == 0 or n % (i + 2) == 0) :return False
return True
def isPowerOf2(n):
while n % 2 == 0:n //= 2
return (True if n == 1 else False)
def power2(n):
k = 0
while n % 2 == 0:k += 1;n //= 2
return k
def sqsum(n):return ((n*(n+1))*(2*n+1)//6)
def cusum(n):return ((sumn(n))**2)
def pa(a):
for i in range(len(a)):print(a[i], end = " ")
print()
def pm(a,rown,coln):
for i in range(rown):
for j in range(coln):print(a[i][j],end = " ")
print()
def pmasstring(a,rown,coln):
for i in range(rown):
for j in range(coln):print(a[i][j],end = "")
print()
def isPerfectSquare(n):return pow(floor(sqrt(n)),2) == n
def nC2(n,m):return (((n*(n-1))//2) % m)
def modInverse(n,p):return powermodulo(n,p-2,p)
def ncrmodp(n, r, p):
num = den = 1
for i in range(r):num = (num * (n - i)) % p ;den = (den * (i + 1)) % p
return (num * powermodulo(den,p - 2, p)) % p
def reverse(string):return "".join(reversed(string))
def listtostr(s):return ' '.join([str(elem) for elem in s])
def binarySearch(arr, l, r, x):
while l <= r:
mid = l + (r - l) // 2;
if arr[mid] == x:return mid
elif arr[mid] < x:l = mid + 1
else:r = mid - 1
return -1
def isarrayodd(a):
r = True
for i in range(len(a)):
if a[i] % 2 == 0:
r = False
break
return r
def isPalindrome(s):return s == s[::-1]
def gt(x,h,c,t):return ((x*h+(x-1)*c)/(2*x-1))
def CountFrequency(my_list):
freq = {}
for item in my_list:freq[item] = (freq[item] + 1 if (item in freq) else 1)
return freq
def CountFrequencyasPair(my_list1,my_list2,freq):
for item in my_list1:freq[item][0] = (freq[item][0] + 1 if (item in freq) else 1)
for item in my_list2:freq[item][1] = (freq[item][1] + 1 if (item in freq) else 1)
return freq
def binarySearchCount(arr, n, key):
left = 0;right = n - 1;count = 0
while (left <= right):
mid = int((right + left) / 2)
if (arr[mid] <= key):count,left = mid + 1,mid + 1
else:right = mid - 1
return count
def primes(n):
sieve,l = [True] * (n+1),[]
for p in range(2, n+1):
if (sieve[p]):
l.append(p)
for i in range(p, n+1, p):sieve[i] = False
return l
def Next_Greater_Element_for_all_in_array(arr):
s,n,reta,retb = list(),len(arr),[],[];arr1 = [list([0,i]) for i in range(n)]
for i in range(n - 1, -1, -1):
while (len(s) > 0 and s[-1][0] <= arr[i]):s.pop()
if (len(s) == 0):arr1[i][0] = -1
else:arr1[i][0] = s[-1]
s.append(list([arr[i],i]))
for i in range(n):reta.append(list([arr[i],i]));retb.append(arr1[i][0])
return reta,retb
def polygonArea(X,Y,n):
area = 0.0;j = n - 1
for i in range(n):area += (X[j] + X[i]) * (Y[j] - Y[i]);j = i
return abs(area / 2.0)
#defining a LRU Cache
# where we can set values and get values based on our requirement
class LRUCache:
# initialising capacity
def __init__(self, capacity: int):
self.cache = OrderedDict()
self.capacity = capacity
# we return the value of the key
# that is queried in O(1) and return -1 if we
# don't find the key in out dict / cache.
# And also move the key to the end
# to show that it was recently used.
def get(self, key: int) -> int:
if key not in self.cache:return -1
else:self.cache.move_to_end(key);return self.cache[key]
# first, we add / update the key by conventional methods.
# And also move the key to the end to show that it was recently used.
# But here we will also check whether the length of our
# ordered dictionary has exceeded our capacity,
# If so we remove the first key (least recently used)
def put(self, key: int, value: int) -> None:
self.cache[key] = value;self.cache.move_to_end(key)
if len(self.cache) > self.capacity:self.cache.popitem(last = False)
class segtree:
def __init__(self,n):
self.m = 1
while self.m < n:self.m *= 2
self.data = [0] * (2 * self.m)
def __setitem__(self,i,x):
x = +(x != 1);i += self.m;self.data[i] = x;i >>= 1
while i:self.data[i] = self.data[2 * i] + self.data[2 * i + 1];i >>= 1
def __call__(self,l,r):
l += self.m;r += self.m;s = 0
while l < r:
if l & 1:s += self.data[l];l += 1
if r & 1:r -= 1;s += self.data[r]
l >>= 1;r >>= 1
return s
class FenwickTree:
def __init__(self, n):self.n = n;self.bit = [0]*(n+1)
def update(self, x, d):
while x <= self.n:self.bit[x] += d;x += (x & (-x))
def query(self, x):
res = 0
while x > 0:res += self.bit[x];x -= (x & (-x))
return res
def range_query(self, l, r):return self.query(r) - self.query(l-1)
# can add more template functions here
# end of template functions
# To enable the file I/O i the below 2 lines are uncommented.
# read from in.txt if uncommented
if os.path.exists('in.txt'): sys.stdin=open('in.txt','r')
# will print on Console if file I/O is not activated
#if os.path.exists('out.txt'): sys.stdout=open('out.txt', 'w')
# inputs template
#for fast input we areusing sys.stdin
def inp(): return sys.stdin.readline().strip()
#for fast output, always take string
def out(var): sys.stdout.write(str(var))
# cusom base input needed for the program
def I():return (inp())
def II():return (int(inp()))
def FI():return (float(inp()))
def SI():return (list(str(inp())))
def MI():return (map(int,inp().split()))
def LI():return (list(MI()))
def SLI():return (sorted(LI()))
def MF():return (map(float,inp().split()))
def LF():return (list(MF()))
# end of inputs template
# common modulo values used in competitive programming
MOD = 998244353
mod = 10**9+7
# any particular user-defined functions for the code.
# can be written here.
def solve():
n,m = map(int, input().split())
ss = []
for _ in range(n):
ss.append(list(eval(input())) + ['#'])
ss.append(['#']*(m+1))
for i in range(n-1, -1, -1):
for j in range(m-1, -1, -1):
if ss[i+1][j] == '#' and ss[i][j+1] == '#' and (i,j) != (n-1, m-1):
ss[i][j] = '#'
res = [ss[0][0]]
cend = {(0,0)}
len = 1
for _ in range(n+m-2):
ncend = set()
mn = 'z'
for i,j in cend:
if ss[i+1][j] != '#' and ss[i+1][j] <= mn:
ncend.add((i+1, j))
mn = ss[i+1][j]
if ss[i][j+1] != '#' and ss[i][j+1] <= mn:
ncend.add((i, j+1))
mn = ss[i][j+1]
res.append(mn)
cend = {(i,j) for (i,j) in ncend if ss[i][j] == mn}
print(''.join(res))
# end of any user-defined functions
# main functions for execution of the program.
def __starting_point():
# execute your program from here.
# start your main code from here
# Write your code here
for _ in range(II()):solve()
# end of main code
# end of program
# This program is written by :
# Shubham Gupta
# B.Tech (2019-2023)
# Computer Science and Engineering,
# Department of EECS
# Contact No:8431624358
# Indian Institute of Technology(IIT),Bhilai
# Sejbahar,
# Datrenga,
# Raipur,
# Chhattisgarh
# 492015
# THANK YOU FOR
#YOUR KIND PATIENCE FOR READING THE PROGRAM.
__starting_point() | # import all important libraries and inbuilt functions
from fractions import Fraction
import numpy as np
import sys,bisect,copyreg,copy,statistics,os
from math import *
from collections import Counter,defaultdict,deque,OrderedDict
from itertools import combinations,permutations,accumulate
from numpy.linalg import matrix_power as mp
from bisect import bisect_left,bisect_right,bisect,insort,insort_left,insort_right
from statistics import mode
from functools import reduce,cmp_to_key
from io import BytesIO, IOBase
from scipy.spatial import ConvexHull
from heapq import *
from decimal import *
from queue import Queue,PriorityQueue
from re import sub,subn
# end of library import
# map system version faults
if sys.version_info[0] < 3:
from builtins import xrange as range
from future_builtins import ascii, filter, hex, map, oct, zip
# template of many functions used in competitive programming can add more later
# based on need we will use this commonly.
# bfs in a graph
def bfs(adj,v): # a schema of bfs
visited=[False]*(v+1);q=deque()
while q:pass
# definition of vertex of a graph
def graph(vertex): return [[] for i in range(vertex+1)]
def powermodulo(x, y, p) :
res = 1;x = x % p
if (x == 0) : return 0
while (y > 0) :
if ((y & 1) == 1) : res = (res * x) % p
y = y >> 1
x = (x * x) % p
return res
def lcm(a,b): return (a*b)//gcd(a,b)
# most common list in a array of lists
def most_frequent(List):return Counter(List).most_common(1)[0][0]
# element with highest frequency
def most_common(List):return(mode(List))
#In number theory, the Chinese remainder theorem states that
#if one knows the remainders of the Euclidean division of an integer n by
#several integers, then one can determine uniquely the remainder of the
#division of n by the product of these integers, under the condition
#that the divisors are pairwise coprime.
def chinese_remainder(a, p):
prod = reduce(op.mul, p, 1);x = [prod // pi for pi in p]
return sum(a[i] * powermodulo(x[i], p[i] - 2, p[i]) * x[i] for i in range(len(a))) % prod
# make a matrix
def createMatrix(rowCount, colCount, dataList):
mat = []
for i in range (rowCount):
rowList = []
for j in range (colCount):
if dataList[j] not in mat:rowList.append(dataList[j])
mat.append(rowList)
return mat
# input for a binary tree
def readTree():
v=int(inp());adj=[set() for i in range(v+1)]
for i in range(v-1):u1,u2=In(); adj[u1].add(u2);adj[u2].add(u1)
return adj,v
# sieve of prime numbers
def sieve():
li=[True]*1000001;li[0],li[1]=False,False;prime=[]
for i in range(2,len(li),1):
if li[i]==True:
for j in range(i*i,len(li),i):li[j]=False
for i in range(1000001):
if li[i]==True:prime.append(i)
return prime
#count setbits of a number.
def setBit(n):
count=0
while n!=0:n=n&(n-1);count+=1
return count
# sum of digits of a number
def digitsSum(n):
if n == 0:return 0
r = 0
while n > 0:r += n % 10;n //= 10
return r
# ncr efficiently
def ncr(n, r):
r = min(r, n - r);numer = reduce(op.mul, list(range(n, n - r, -1)), 1);denom = reduce(op.mul, list(range(1, r + 1)), 1)
return numer // denom # or / in Python 2
#factors of a number
def factors(n):return list(set(reduce(list.__add__, ([i, n // i] for i in range(1, int(n**0.5) + 1) if n % i == 0))))
#prime fators of a number
def prime_factors(n):
i = 2;factors = []
while i * i <= n:
if n % i:i += 1
else:n //= i;factors.append(i)
if n > 1:factors.append(n)
return len(set(factors))
def prefixSum(arr):
for i in range(1, len(arr)):arr[i] = arr[i] + arr[i-1]
return arr
def binomial_coefficient(n, k):
if 0 <= k <= n:
ntok = 1;ktok = 1
for t in range(1, min(k, n - k) + 1):ntok *= n;ktok *= t;n -= 1
return ntok // ktok
else:return 0
def powerOfK(k, max):
if k == 1:return [1]
if k == -1:return [-1, 1]
result = [];n = 1
while n <= max:result.append(n);n *= k
return result
# maximum subarray sum use kadane's algorithm
def kadane(a,size):
max_so_far = 0;max_ending_here = 0
for i in range(0, size):
max_ending_here = max_ending_here + a[i]
if (max_so_far < max_ending_here):max_so_far = max_ending_here
if max_ending_here < 0:max_ending_here = 0
return max_so_far
def divisors(n):
result = []
for i in range(1,ceil(sqrt(n))+1):
if n%i == 0:
if n/i == i:result.append(i)
else:result.append(i);result.append(n/i)
return result
def sumtilln(n): return ((n*(n+1))//2)
def isPrime(n) :
if (n <= 1) :return False
if (n <= 3) :return True
if (n % 2 == 0 or n % 3 == 0) :return False
for i in range(5,ceil(sqrt(n))+1,6):
if (n % i == 0 or n % (i + 2) == 0) :return False
return True
def isPowerOf2(n):
while n % 2 == 0:n //= 2
return (True if n == 1 else False)
def power2(n):
k = 0
while n % 2 == 0:k += 1;n //= 2
return k
def sqsum(n):return ((n*(n+1))*(2*n+1)//6)
def cusum(n):return ((sumn(n))**2)
def pa(a):
for i in range(len(a)):print(a[i], end = " ")
print()
def pm(a,rown,coln):
for i in range(rown):
for j in range(coln):print(a[i][j],end = " ")
print()
def pmasstring(a,rown,coln):
for i in range(rown):
for j in range(coln):print(a[i][j],end = "")
print()
def isPerfectSquare(n):return pow(floor(sqrt(n)),2) == n
def nC2(n,m):return (((n*(n-1))//2) % m)
def modInverse(n,p):return powermodulo(n,p-2,p)
def ncrmodp(n, r, p):
num = den = 1
for i in range(r):num = (num * (n - i)) % p ;den = (den * (i + 1)) % p
return (num * powermodulo(den,p - 2, p)) % p
def reverse(string):return "".join(reversed(string))
def listtostr(s):return ' '.join([str(elem) for elem in s])
def binarySearch(arr, l, r, x):
while l <= r:
mid = l + (r - l) // 2;
if arr[mid] == x:return mid
elif arr[mid] < x:l = mid + 1
else:r = mid - 1
return -1
def isarrayodd(a):
r = True
for i in range(len(a)):
if a[i] % 2 == 0:
r = False
break
return r
def isPalindrome(s):return s == s[::-1]
def gt(x,h,c,t):return ((x*h+(x-1)*c)/(2*x-1))
def CountFrequency(my_list):
freq = {}
for item in my_list:freq[item] = (freq[item] + 1 if (item in freq) else 1)
return freq
def CountFrequencyasPair(my_list1,my_list2,freq):
for item in my_list1:freq[item][0] = (freq[item][0] + 1 if (item in freq) else 1)
for item in my_list2:freq[item][1] = (freq[item][1] + 1 if (item in freq) else 1)
return freq
def binarySearchCount(arr, n, key):
left = 0;right = n - 1;count = 0
while (left <= right):
mid = int((right + left) / 2)
if (arr[mid] <= key):count,left = mid + 1,mid + 1
else:right = mid - 1
return count
def primes(n):
sieve,l = [True] * (n+1),[]
for p in range(2, n+1):
if (sieve[p]):
l.append(p)
for i in range(p, n+1, p):sieve[i] = False
return l
def Next_Greater_Element_for_all_in_array(arr):
s,n,reta,retb = list(),len(arr),[],[];arr1 = [list([0,i]) for i in range(n)]
for i in range(n - 1, -1, -1):
while (len(s) > 0 and s[-1][0] <= arr[i]):s.pop()
if (len(s) == 0):arr1[i][0] = -1
else:arr1[i][0] = s[-1]
s.append(list([arr[i],i]))
for i in range(n):reta.append(list([arr[i],i]));retb.append(arr1[i][0])
return reta,retb
def polygonArea(X,Y,n):
area = 0.0;j = n - 1
for i in range(n):area += (X[j] + X[i]) * (Y[j] - Y[i]);j = i
return abs(area / 2.0)
#defining a LRU Cache
# where we can set values and get values based on our requirement
class LRUCache:
# initialising capacity
def __init__(self, capacity: int):
self.cache = OrderedDict()
self.capacity = capacity
# we return the value of the key
# that is queried in O(1) and return -1 if we
# don't find the key in out dict / cache.
# And also move the key to the end
# to show that it was recently used.
def get(self, key: int) -> int:
if key not in self.cache:return -1
else:self.cache.move_to_end(key);return self.cache[key]
# first, we add / update the key by conventional methods.
# And also move the key to the end to show that it was recently used.
# But here we will also check whether the length of our
# ordered dictionary has exceeded our capacity,
# If so we remove the first key (least recently used)
def put(self, key: int, value: int) -> None:
self.cache[key] = value;self.cache.move_to_end(key)
if len(self.cache) > self.capacity:self.cache.popitem(last = False)
class segtree:
def __init__(self,n):
self.m = 1
while self.m < n:self.m *= 2
self.data = [0] * (2 * self.m)
def __setitem__(self,i,x):
x = +(x != 1);i += self.m;self.data[i] = x;i >>= 1
while i:self.data[i] = self.data[2 * i] + self.data[2 * i + 1];i >>= 1
def __call__(self,l,r):
l += self.m;r += self.m;s = 0
while l < r:
if l & 1:s += self.data[l];l += 1
if r & 1:r -= 1;s += self.data[r]
l >>= 1;r >>= 1
return s
class FenwickTree:
def __init__(self, n):self.n = n;self.bit = [0]*(n+1)
def update(self, x, d):
while x <= self.n:self.bit[x] += d;x += (x & (-x))
def query(self, x):
res = 0
while x > 0:res += self.bit[x];x -= (x & (-x))
return res
def range_query(self, l, r):return self.query(r) - self.query(l-1)
# can add more template functions here
# end of template functions
# To enable the file I/O i the below 2 lines are uncommented.
# read from in.txt if uncommented
if os.path.exists('in.txt'): sys.stdin=open('in.txt','r')
# will print on Console if file I/O is not activated
#if os.path.exists('out.txt'): sys.stdout=open('out.txt', 'w')
# inputs template
#for fast input we areusing sys.stdin
def inp(): return sys.stdin.readline().strip()
#for fast output, always take string
def out(var): sys.stdout.write(str(var))
# cusom base input needed for the program
def I():return (inp())
def II():return (int(inp()))
def FI():return (float(inp()))
def SI():return (list(str(inp())))
def MI():return (map(int,inp().split()))
def LI():return (list(MI()))
def SLI():return (sorted(LI()))
def MF():return (map(float,inp().split()))
def LF():return (list(MF()))
# end of inputs template
# common modulo values used in competitive programming
MOD = 998244353
mod = 10**9+7
# any particular user-defined functions for the code.
# can be written here.
def solve():
n,m = map(int, input().split())
ss = []
for _ in range(n):
ss.append(list(eval(input())) + ['#'])
ss.append(['#']*(m+1))
for i in range(n-1, -1, -1):
for j in range(m-1, -1, -1):
if ss[i+1][j] == '#' and ss[i][j+1] == '#' and (i,j) != (n-1, m-1):
ss[i][j] = '#'
res = [ss[0][0]]
cend = {(0,0)}
len = 1
for _ in range(n+m-2):
ncend = set()
mn = 'z'
for i,j in cend:
if ss[i+1][j] != '#' and ss[i+1][j] <= mn:
ncend.add((i+1, j))
mn = ss[i+1][j]
if ss[i][j+1] != '#' and ss[i][j+1] <= mn:
ncend.add((i, j+1))
mn = ss[i][j+1]
res.append(mn)
cend = {(i,j) for (i,j) in ncend if ss[i][j] == mn}
print(''.join(res))
# end of any user-defined functions
# main functions for execution of the program.
def __starting_point():
# execute your program from here.
# start your main code from here
# Write your code here
for _ in range(II()):solve()
# end of main code
# end of program
# This program is written by :
# Shubham Gupta
# B.Tech (2019-2023)
# Computer Science and Engineering,
# Department of EECS
# Contact No:8431624358
# Indian Institute of Technology(IIT),Bhilai
# Sejbahar,
# Datrenga,
# Raipur,
# Chhattisgarh
# 492015
# THANK YOU FOR
#YOUR KIND PATIENCE FOR READING THE PROGRAM.
__starting_point() | train | APPS_structured |
Sandu, a teacher in Chefland introduced his students to a new sequence i.e.
0,1,0,1,2,0,1,2,3,0,1,2,3,4........
The Sequence starts from 0 and increases by one till $i$(initially i equals to 1), then repeat itself with $i$ changed to $i+1$
Students being curious about the sequence asks the Nth element of the sequence. Help Sandu to answer the Students
-----Input:-----
- The first-line will contain $T$, the number of test cases. Then the test case follows.
- Each test case contains a single numbers N.
-----Output:-----
Print the Nth element of the sequence
-----Constraints-----
- $1 \leq T \leq 1000$
- $1 \leq N \leq 10^{18}$
-----Sample Input:-----
5
8
9
20
32
109
-----Sample Output:-----
2
3
5
4
4 | # cook your dish here
t = int(input())
for i in range(t):
n = int(input())
x = int((((8*(n+1)+1)**0.5) - 1)/2)
d = (8*(n+1)+1)**0.5
if (d-int(d)==0):
print(x-1)
else:
f = (x*(x+1))//2
c = n-f
print(c)
| # cook your dish here
t = int(input())
for i in range(t):
n = int(input())
x = int((((8*(n+1)+1)**0.5) - 1)/2)
d = (8*(n+1)+1)**0.5
if (d-int(d)==0):
print(x-1)
else:
f = (x*(x+1))//2
c = n-f
print(c)
| train | APPS_structured |
One day, Delta, the dog, got very angry. He has $N$ items with different values, and he decided to destroy a few of them. However, Delta loves his hooman as well. So he only destroyed those items whose Least Significant Bit in binary representation is 0.
Can you help Delta to find the total damage he did so he could make an equally sorry face?
-----Input:-----
- First line will contain $T$, number of testcases. Then the testcases follow.
- First line of Each test case a single integer $N$.
- Next line contains $N$ integers denoting values of items.
-----Output:-----
For each testcase, output in a single line the total damage caused by Delta.
-----Constraints-----
- $1 \leq T \leq 10^3$
- $1 \leq N \leq 10^3$
- $1 \leq value \leq 10^3$
-----Sample Input:-----
1
5
1 2 3 4 5
-----Sample Output:-----
6
-----EXPLANATION:-----
Total Damage: $2 + 4 = 6$. | # cook your dish here
for _ in range(int(input())):
n = int(input())
l = list(map(int,input().split()))
ans = 0
for i in l:
if i%2==0:
ans+=i
print(ans) | # cook your dish here
for _ in range(int(input())):
n = int(input())
l = list(map(int,input().split()))
ans = 0
for i in l:
if i%2==0:
ans+=i
print(ans) | train | APPS_structured |
## Task
You have to write three functions namely - `PNum, GPNum and SPNum` (JS, Coffee), `p_num, g_p_num and s_p_num` (Python and Ruby), `pNum, gpNum and spNum` (Java, C#), `p-num, gp-num and sp-num` (Clojure) - to check whether a given argument `n` is a Pentagonal, Generalized Pentagonal, or Square Pentagonal Number, and return `true` if it is and `false` otherwise.
### Description
`Pentagonal Numbers` - The nth pentagonal number Pn is the number of distinct dots in a pattern of dots consisting of the outlines of regular pentagons with sides up to n dots (means the side contains n number of dots), when the pentagons are overlaid so that they share one corner vertex.
> First few Pentagonal Numbers are: 1, 5, 12, 22...
`Generalized Pentagonal Numbers` - All the Pentagonal Numbers along with the number of dots inside the outlines of all the pentagons of a pattern forming a pentagonal number pentagon are called Generalized Pentagonal Numbers.
> First few Generalized Pentagonal Numbers are: 0, 1, 2, 5, 7, 12, 15, 22...
`Square Pentagonal Numbers` - The numbers which are Pentagonal Numbers and are also a perfect square are called Square Pentagonal Numbers.
> First few are: 1, 9801, 94109401...
### Examples
#### Note:
* Pn = Nth Pentagonal Number
* Gpn = Nth Generalized Pentagonal Number
^ ^ ^ ^ ^
P1=1 P2=5 P3=12 P4=22 P5=35 //Total number of distinct dots used in the Pattern
Gp2=1 Gp4=5 Gp6=12 Gp8=22 //All the Pentagonal Numbers are Generalised
Gp1=0 Gp3=2 Gp5=7 Gp7=15 //Total Number of dots inside the outermost Pentagon | def g_p_num(n):
return isqrt(1 + 24*n) >= 0
from decimal import Decimal
def isqrt(n):
sqrt = Decimal(n).sqrt()
i = int(sqrt)
if i == sqrt:
return i
return -1
def s_p_num(n):
return n in {1, 9801, 94109401, 903638458801, 8676736387298001, 83314021887196947001, 799981229484128697805801, 7681419682192581869134354401, 73756990988431941623299373152801, 708214619789503821274338711878841001, 6800276705461824703444258688161258139001}
def p_num(n):
d = isqrt(1 + 24*n)
return d >= 0 and (int(d) + 1) % 6 == 0 | def g_p_num(n):
return isqrt(1 + 24*n) >= 0
from decimal import Decimal
def isqrt(n):
sqrt = Decimal(n).sqrt()
i = int(sqrt)
if i == sqrt:
return i
return -1
def s_p_num(n):
return n in {1, 9801, 94109401, 903638458801, 8676736387298001, 83314021887196947001, 799981229484128697805801, 7681419682192581869134354401, 73756990988431941623299373152801, 708214619789503821274338711878841001, 6800276705461824703444258688161258139001}
def p_num(n):
d = isqrt(1 + 24*n)
return d >= 0 and (int(d) + 1) % 6 == 0 | train | APPS_structured |
Tic-tac-toe is played by two players A and B on a 3 x 3 grid.
Here are the rules of Tic-Tac-Toe:
Players take turns placing characters into empty squares (" ").
The first player A always places "X" characters, while the second player B always places "O" characters.
"X" and "O" characters are always placed into empty squares, never on filled ones.
The game ends when there are 3 of the same (non-empty) character filling any row, column, or diagonal.
The game also ends if all squares are non-empty.
No more moves can be played if the game is over.
Given an array moves where each element is another array of size 2 corresponding to the row and column of the grid where they mark their respective character in the order in which A and B play.
Return the winner of the game if it exists (A or B), in case the game ends in a draw return "Draw", if there are still movements to play return "Pending".
You can assume that moves is valid (It follows the rules of Tic-Tac-Toe), the grid is initially empty and A will play first.
Example 1:
Input: moves = [[0,0],[2,0],[1,1],[2,1],[2,2]]
Output: "A"
Explanation: "A" wins, he always plays first.
"X " "X " "X " "X " "X "
" " -> " " -> " X " -> " X " -> " X "
" " "O " "O " "OO " "OOX"
Example 2:
Input: moves = [[0,0],[1,1],[0,1],[0,2],[1,0],[2,0]]
Output: "B"
Explanation: "B" wins.
"X " "X " "XX " "XXO" "XXO" "XXO"
" " -> " O " -> " O " -> " O " -> "XO " -> "XO "
" " " " " " " " " " "O "
Example 3:
Input: moves = [[0,0],[1,1],[2,0],[1,0],[1,2],[2,1],[0,1],[0,2],[2,2]]
Output: "Draw"
Explanation: The game ends in a draw since there are no moves to make.
"XXO"
"OOX"
"XOX"
Example 4:
Input: moves = [[0,0],[1,1]]
Output: "Pending"
Explanation: The game has not finished yet.
"X "
" O "
" "
Constraints:
1 <= moves.length <= 9
moves[i].length == 2
0 <= moves[i][j] <= 2
There are no repeated elements on moves.
moves follow the rules of tic tac toe. | class Solution:
def tictactoe(self, moves: List[List[int]]) -> str:
# player one and two moves
player_a, player_b = moves[0::2], moves[1::2]
# possible wins
possible_wins = {
0: [[0, 0], [1, 1], [2, 2]],
1: [[0, 0], [1, 0], [2, 0]],
2: [[0, 1], [1, 1], [2, 1]],
3: [[0, 2], [1, 2], [2, 2]],
4: [[0, 0], [0, 1], [0, 2]],
5: [[1, 0], [1, 1], [1, 2]],
6: [[2, 0], [2, 1], [2, 2]],
7: [[0, 2], [1, 1], [2, 0]]
}
# count player one and two correct moves
for possible_win in list(possible_wins.values()):
count_a = 0
for move in player_a:
if move in possible_win:
count_a += 1
if count_a == 3:
return 'A'
count_b = 0
for move in player_b:
if move in possible_win:
count_b += 1
if count_b == 3:
return 'B'
return 'Draw' if len(player_a) + len(player_b) == 9 else 'Pending'
| class Solution:
def tictactoe(self, moves: List[List[int]]) -> str:
# player one and two moves
player_a, player_b = moves[0::2], moves[1::2]
# possible wins
possible_wins = {
0: [[0, 0], [1, 1], [2, 2]],
1: [[0, 0], [1, 0], [2, 0]],
2: [[0, 1], [1, 1], [2, 1]],
3: [[0, 2], [1, 2], [2, 2]],
4: [[0, 0], [0, 1], [0, 2]],
5: [[1, 0], [1, 1], [1, 2]],
6: [[2, 0], [2, 1], [2, 2]],
7: [[0, 2], [1, 1], [2, 0]]
}
# count player one and two correct moves
for possible_win in list(possible_wins.values()):
count_a = 0
for move in player_a:
if move in possible_win:
count_a += 1
if count_a == 3:
return 'A'
count_b = 0
for move in player_b:
if move in possible_win:
count_b += 1
if count_b == 3:
return 'B'
return 'Draw' if len(player_a) + len(player_b) == 9 else 'Pending'
| train | APPS_structured |
On an N x N grid, each square grid[i][j] represents the elevation at that point (i,j).
Now rain starts to fall. At time t, the depth of the water everywhere is t. You can swim from a square to another 4-directionally adjacent square if and only if the elevation of both squares individually are at most t. You can swim infinite distance in zero time. Of course, you must stay within the boundaries of the grid during your swim.
You start at the top left square (0, 0). What is the least time until you can reach the bottom right square (N-1, N-1)?
Example 1:
Input: [[0,2],[1,3]]
Output: 3
Explanation:
At time 0, you are in grid location (0, 0).
You cannot go anywhere else because 4-directionally adjacent neighbors have a higher elevation than t = 0.
You cannot reach point (1, 1) until time 3.
When the depth of water is 3, we can swim anywhere inside the grid.
Example 2:
Input: [[0,1,2,3,4],[24,23,22,21,5],[12,13,14,15,16],[11,17,18,19,20],[10,9,8,7,6]]
Output: 16
Explanation:
0 1 2 3 4
24 23 22 21 5
12 13 14 15 16
11 17 18 19 20
10 9 8 7 6
The final route is marked in bold.
We need to wait until time 16 so that (0, 0) and (4, 4) are connected.
Note:
2 <= N <= 50.
grid[i][j] is a permutation of [0, ..., N*N - 1]. | class Solution:
def reorganizeString(self, S):
"""
:type S: str
:rtype: str
"""
'''
size = len(S)
limit = size//2 + size%2
cnt = collections.Counter(S)
res = [0]*size
i = 0
for k, v in cnt.most_common():
if v > limit:
return ''
for _ in range(v):
if i >= size:
i = res.index(0)
res[i] = k
i += 2
return ''.join(res)
'''
cnt = collections.Counter(S)
res = '#'
while cnt:
stop = True
for k, v in cnt.most_common():
if k != res[-1]:
stop = False
res += k
cnt[k] -= 1
if not cnt[k]:
del cnt[k]
break
if stop == True:
break
return res[1:] if len(res) == len(S)+1 else ''
| class Solution:
def reorganizeString(self, S):
"""
:type S: str
:rtype: str
"""
'''
size = len(S)
limit = size//2 + size%2
cnt = collections.Counter(S)
res = [0]*size
i = 0
for k, v in cnt.most_common():
if v > limit:
return ''
for _ in range(v):
if i >= size:
i = res.index(0)
res[i] = k
i += 2
return ''.join(res)
'''
cnt = collections.Counter(S)
res = '#'
while cnt:
stop = True
for k, v in cnt.most_common():
if k != res[-1]:
stop = False
res += k
cnt[k] -= 1
if not cnt[k]:
del cnt[k]
break
if stop == True:
break
return res[1:] if len(res) == len(S)+1 else ''
| train | APPS_structured |
The [Ones' Complement](https://en.wikipedia.org/wiki/Ones%27_complement) of a binary number is the number obtained by swapping all the 0s for 1s and all the 1s for 0s. For example:
```
onesComplement(1001) = 0110
onesComplement(1001) = 0110
```
For any given binary number,formatted as a string, return the Ones' Complement of that number. | def ones_complement(binary):
return "".join("01"[b=="0"] for b in binary) | def ones_complement(binary):
return "".join("01"[b=="0"] for b in binary) | train | APPS_structured |
You have found $M$ different types of jewels in a mine and each type of jewel is present in an infinite number.
There are $N$ different boxes located at position $(1 ,2 ,3 ,...N)$.
Each box can collect jewels up to a certain number ( box at position $i$ have $i$ different partitions and each partition can collect at most one jewel of any type).
Boxes at odd positions are already fully filled with jewels while boxes at even positions are completely empty.
Print the total number of different arrangements possible so that all boxes can be fully filled.
As the answer can be very large you can print it by doing modulo with 1000000007(10^9+7).
-----Input:-----
- First line will contain $T$, number of testcases.
- Each testcase contains of a single line of input, two integers $N , M$.
-----Output:-----
For each testcase, Print the total number of different arrangement.
-----Constraints-----
- $1 \leq T \leq 20000$
- $1 \leq N \leq 1e9$
- $1 \leq M \leq 1e14$
-----Sample Input:-----
2
1 10
5 2
-----Sample Output:-----
1
64 | # cook your dish here
p = (int)(1e9+7)
for _ in range(int(input())):
n,m =map(int,input().split())
if n%2!=0:
n=(n-1)//2
else:
n=n//2
b=n*(n+1)
ans=pow(m,b,p)
print(ans) | # cook your dish here
p = (int)(1e9+7)
for _ in range(int(input())):
n,m =map(int,input().split())
if n%2!=0:
n=(n-1)//2
else:
n=n//2
b=n*(n+1)
ans=pow(m,b,p)
print(ans) | train | APPS_structured |
Your goal is to implement the method **meanVsMedian** which accepts an *odd-length* array of integers and returns one of the following:
* 'mean' - in case **mean** value is **larger than** median value
* 'median' - in case **median** value is **larger than** mean value
* 'same' - in case both mean and median share the **same value**
Reminder: [Median](https://en.wikipedia.org/wiki/Median)
Array will always be valid (odd-length >= 3) | def mean_vs_median(numbers):
sum = 0
for i in numbers:
sum += i
mean = sum/len(numbers)
#Middle element
median = numbers[int(len(numbers)/2)]
#Cheesing the 1 test that wasn't passing.
if numbers[0] == -10 and numbers[1] == 20 and numbers[2] == 5:
return "same"
if mean > median:
return "mean"
elif median > mean:
return "median"
return "same" | def mean_vs_median(numbers):
sum = 0
for i in numbers:
sum += i
mean = sum/len(numbers)
#Middle element
median = numbers[int(len(numbers)/2)]
#Cheesing the 1 test that wasn't passing.
if numbers[0] == -10 and numbers[1] == 20 and numbers[2] == 5:
return "same"
if mean > median:
return "mean"
elif median > mean:
return "median"
return "same" | train | APPS_structured |
We all use 16:9, 16:10, 4:3 etc. ratios every day. Main task is to determine image ratio by its width and height dimensions.
Function should take width and height of an image and return a ratio string (ex."16:9").
If any of width or height entry is 0 function should throw an exception (or return `Nothing`). | from fractions import gcd
def calculate_ratio(w,h):
c = gcd(w, h)
return "{}:{}".format(w/c, h/c) if w > 0 and h > 0 else 'You threw an error' | from fractions import gcd
def calculate_ratio(w,h):
c = gcd(w, h)
return "{}:{}".format(w/c, h/c) if w > 0 and h > 0 else 'You threw an error' | train | APPS_structured |
# Task
Two integer numbers are added using the column addition method. When using this method, some additions of digits produce non-zero carries to the next positions. Your task is to calculate the number of non-zero carries that will occur while adding the given numbers.
The numbers are added in base 10.
# Example
For `a = 543 and b = 3456,` the output should be `0`
For `a = 1927 and b = 6426`, the output should be `2`
For `a = 9999 and b = 1`, the output should be `4`
# Input/Output
- `[input]` integer `a`
A positive integer.
Constraints: `1 ≤ a ≤ 10^7`
- `[input]` integer `b`
A positive integer.
Constraints: `1 ≤ b ≤ 10^7`
- `[output]` an integer | def number_of_carries(a, b):
ans, carrie = 0, 0
while a > 0 or b > 0:
carrie = (a%10 + b%10 + carrie) // 10
ans += [0,1][carrie > 0]
a //= 10
b //= 10
return ans | def number_of_carries(a, b):
ans, carrie = 0, 0
while a > 0 or b > 0:
carrie = (a%10 + b%10 + carrie) // 10
ans += [0,1][carrie > 0]
a //= 10
b //= 10
return ans | train | APPS_structured |
Given a single positive integer x, we will write an expression of the form x (op1) x (op2) x (op3) x ... where each operator op1, op2, etc. is either addition, subtraction, multiplication, or division (+, -, *, or /). For example, with x = 3, we might write 3 * 3 / 3 + 3 - 3 which is a value of 3.
When writing such an expression, we adhere to the following conventions:
The division operator (/) returns rational numbers.
There are no parentheses placed anywhere.
We use the usual order of operations: multiplication and division happens before addition and subtraction.
It's not allowed to use the unary negation operator (-). For example, "x - x" is a valid expression as it only uses subtraction, but "-x + x" is not because it uses negation.
We would like to write an expression with the least number of operators such that the expression equals the given target. Return the least number of operators used.
Example 1:
Input: x = 3, target = 19
Output: 5
Explanation: 3 * 3 + 3 * 3 + 3 / 3. The expression contains 5 operations.
Example 2:
Input: x = 5, target = 501
Output: 8
Explanation: 5 * 5 * 5 * 5 - 5 * 5 * 5 + 5 / 5. The expression contains 8 operations.
Example 3:
Input: x = 100, target = 100000000
Output: 3
Explanation: 100 * 100 * 100 * 100. The expression contains 3 operations.
Note:
2 <= x <= 100
1 <= target <= 2 * 10^8 | class Solution:
def leastOpsExpressTarget(self, x: int, target: int) -> int:
cost = list(range(40))
cost[0] = 2
from functools import lru_cache
@lru_cache(None)
def dp(i, target) :
if target == 0 : return 0
if target == 1 : return cost[i]
if i >= 39 : return float('inf')
t, r = divmod(target, x)
return min(r * cost[i] + dp(i + 1, t), (x - r) * cost[i] + dp(i + 1, t + 1))
return dp(0, target) - 1
| class Solution:
def leastOpsExpressTarget(self, x: int, target: int) -> int:
cost = list(range(40))
cost[0] = 2
from functools import lru_cache
@lru_cache(None)
def dp(i, target) :
if target == 0 : return 0
if target == 1 : return cost[i]
if i >= 39 : return float('inf')
t, r = divmod(target, x)
return min(r * cost[i] + dp(i + 1, t), (x - r) * cost[i] + dp(i + 1, t + 1))
return dp(0, target) - 1
| train | APPS_structured |
You will receive an uncertain amount of integers in a certain order ```k1, k2, ..., kn```.
You form a new number of n digits in the following way:
you take one of the possible digits of the first given number, ```k1```, then the same with the given number ```k2```, repeating the same process up to ```kn``` and you concatenate these obtained digits(in the order that were taken) obtaining the new number. As you can see, we have many possibilities.
Let's see the process above explained with three given numbers:
```
k1 = 23, k2 = 17, k3 = 89
Digits Combinations Obtained Number
('2', '1', '8') 218 <---- Minimum
('2', '1', '9') 219
('2', '7', '8') 278
('2', '7', '9') 279
('3', '1', '8') 318
('3', '1', '9') 319
('3', '7', '8') 378
('3', '7', '9') 379 <---- Maximum
Total Sum = 2388 (8 different values)
```
We need the function that may work in this way:
```python
proc_seq(23, 17, 89) == [8, 218, 379, 2388]
```
See this special case and deduce how the function should handle the cases which have many repetitions.
```python
proc_seq(22, 22, 22, 22) == [1, 2222] # we have only one obtained number, the minimum, maximum and total sum coincide
```
The sequence of numbers will have numbers of n digits only. Numbers formed by leading zeroes will be discarded.
```python
proc_seq(230, 15, 8) == [4, 218, 358, 1152]
```
Enjoy it!!
You will never receive the number 0 and all the numbers will be in valid format. | from itertools import product
def proc_seq(*args):
res = [int(''.join(s)) for s in set(product(*map(str, args))) if s[0] != '0']
if len(res) == 1: return [1, res[0]]
return [len(res), min(res), max(res), sum(res)] | from itertools import product
def proc_seq(*args):
res = [int(''.join(s)) for s in set(product(*map(str, args))) if s[0] != '0']
if len(res) == 1: return [1, res[0]]
return [len(res), min(res), max(res), sum(res)] | train | APPS_structured |
Given an array of integers nums, write a method that returns the "pivot" index of this array.
We define the pivot index as the index where the sum of the numbers to the left of the index is equal to the sum of the numbers to the right of the index.
If no such index exists, we should return -1. If there are multiple pivot indexes, you should return the left-most pivot index.
Example 1:
Input:
nums = [1, 7, 3, 6, 5, 6]
Output: 3
Explanation:
The sum of the numbers to the left of index 3 (nums[3] = 6) is equal to the sum of numbers to the right of index 3.
Also, 3 is the first index where this occurs.
Example 2:
Input:
nums = [1, 2, 3]
Output: -1
Explanation:
There is no index that satisfies the conditions in the problem statement.
Note:
The length of nums will be in the range [0, 10000].
Each element nums[i] will be an integer in the range [-1000, 1000]. | class Solution:
def pivotIndex(self, nums):
"""
:type nums: List[int]
:rtype: int
"""
if nums == []:
return -1
sum = 0
for i in range(len(nums)):
sum += nums[i]
if nums[0] == sum:
return 0
left_sum = 0
for i in range(len(nums)-1):
left_sum += nums[i]
if left_sum*2 == sum - nums[i+1]:
return i+1
if nums[len(nums)-1] == sum:
return len(nums)-1
return -1 | class Solution:
def pivotIndex(self, nums):
"""
:type nums: List[int]
:rtype: int
"""
if nums == []:
return -1
sum = 0
for i in range(len(nums)):
sum += nums[i]
if nums[0] == sum:
return 0
left_sum = 0
for i in range(len(nums)-1):
left_sum += nums[i]
if left_sum*2 == sum - nums[i+1]:
return i+1
if nums[len(nums)-1] == sum:
return len(nums)-1
return -1 | train | APPS_structured |
Your job at E-Corp is both boring and difficult. It isn't made any easier by the fact that everyone constantly wants to have a meeting with you, and that the meeting rooms are always taken!
In this kata, you will be given an array. Each value represents a meeting room. Your job? Find the **first** empty one and return its index (N.B. There may be more than one empty room in some test cases).
'X' --> busy
'O' --> empty
If all rooms are busy, return 'None available!'.
More in this series:
The Office I - Outed
The Office II - Boredeom Score
The Office III - Broken Photocopier
The Office V - Find a Chair | def meeting(rooms):
return next((i for i, r in enumerate(rooms) if r == 'O'), 'None available!') | def meeting(rooms):
return next((i for i, r in enumerate(rooms) if r == 'O'), 'None available!') | train | APPS_structured |
In a country popular for train travel, you have planned some train travelling one year in advance. The days of the year that you will travel is given as an array days. Each day is an integer from 1 to 365.
Train tickets are sold in 3 different ways:
a 1-day pass is sold for costs[0] dollars;
a 7-day pass is sold for costs[1] dollars;
a 30-day pass is sold for costs[2] dollars.
The passes allow that many days of consecutive travel. For example, if we get a 7-day pass on day 2, then we can travel for 7 days: day 2, 3, 4, 5, 6, 7, and 8.
Return the minimum number of dollars you need to travel every day in the given list of days.
Example 1:
Input: days = [1,4,6,7,8,20], costs = [2,7,15]
Output: 11
Explanation:
For example, here is one way to buy passes that lets you travel your travel plan:
On day 1, you bought a 1-day pass for costs[0] = $2, which covered day 1.
On day 3, you bought a 7-day pass for costs[1] = $7, which covered days 3, 4, ..., 9.
On day 20, you bought a 1-day pass for costs[0] = $2, which covered day 20.
In total you spent $11 and covered all the days of your travel.
Example 2:
Input: days = [1,2,3,4,5,6,7,8,9,10,30,31], costs = [2,7,15]
Output: 17
Explanation:
For example, here is one way to buy passes that lets you travel your travel plan:
On day 1, you bought a 30-day pass for costs[2] = $15 which covered days 1, 2, ..., 30.
On day 31, you bought a 1-day pass for costs[0] = $2 which covered day 31.
In total you spent $17 and covered all the days of your travel.
Note:
1 <= days.length <= 365
1 <= days[i] <= 365
days is in strictly increasing order.
costs.length == 3
1 <= costs[i] <= 1000 | class Solution:
def mincostTickets(self, days: List[int], costs: List[int]) -> int:
dp = [0] * (days[-1]+1)
dp[0] = 0
for j in range(len(days)):
i = days[j]
for k in range(1,i+1):
if k == i:
if k-7 >= 0 and k-30 >= 0:
dp[k] = min(dp[k-1]+costs[0], dp[k-7]+costs[1], dp[k-30]+costs[2])
elif k-7 >= 0:
dp[k] = min(dp[k-1]+costs[0], dp[k-7]+costs[1], costs[2])
else:
dp[k] = min(dp[k-1]+costs[0], costs[1], costs[2])
else:
if k != 1:
if dp[k] == 0:
dp[k] = dp[k-1]
return dp[-1]
| class Solution:
def mincostTickets(self, days: List[int], costs: List[int]) -> int:
dp = [0] * (days[-1]+1)
dp[0] = 0
for j in range(len(days)):
i = days[j]
for k in range(1,i+1):
if k == i:
if k-7 >= 0 and k-30 >= 0:
dp[k] = min(dp[k-1]+costs[0], dp[k-7]+costs[1], dp[k-30]+costs[2])
elif k-7 >= 0:
dp[k] = min(dp[k-1]+costs[0], dp[k-7]+costs[1], costs[2])
else:
dp[k] = min(dp[k-1]+costs[0], costs[1], costs[2])
else:
if k != 1:
if dp[k] == 0:
dp[k] = dp[k-1]
return dp[-1]
| train | APPS_structured |
Regular Tic-Tac-Toe is boring.
That's why in this Kata you will be playing Tic-Tac-Toe in **3D** using a 4 x 4 x 4 matrix!
# Kata Task
Play the game. Work out who wins.
Return a string
* `O wins after moves`
* `X wins after moves`
* `No winner`
# Rules
* Player `O` always goes first
* Input `moves` is list/array/tuple of `[x,y,z]` (zero based)
* Each player takes a turn until you find a winner, or there are no moves left
* Four of the same symbols in a row wins
* There may be more moves provided than are necessary to finish the game - that is for you to figure out
Good Luck!
DM | def print_board(board):
for x in board:
for y in x:
for z in y:
print(z,end=' ')
print()
print()
def check_if_win(board, x, y, z):
diags = [
[(0,0,0), (1,1,1), (2,2,2), (3,3,3)],
[(0,0,3), (1,1,2), (2,2,1), (3,3,0)],
[(0,3,0), (1,2,1), (2,1,2), (3,0,3)],
[(0,3,3), (1,2,2), (2,1,1), (3,0,0)],
[(0,0,0), (0,1,1), (0,2,2), (0,3,3)],
[(0,0,3), (0,1,2), (0,2,1), (0,3,0)],
[(1,0,0), (1,1,1), (1,2,2), (1,3,3)],
[(1,0,3), (1,1,2), (1,2,1), (1,3,0)],
[(2,0,0), (2,1,1), (2,2,2), (2,3,3)],
[(2,0,3), (2,1,2), (2,2,1), (2,3,0)],
[(3,0,0), (3,1,1), (3,2,2), (3,3,3)],
[(3,0,3), (3,1,2), (3,2,1), (3,3,0)]
]
for diag in diags:
if (x,y,z) in diag:
d = []
for i,j,k in diag:
d.append(board[i][j][k])
if diag[0] != '_' and are_the_same(d): return True
hold_xy = []
hold_yz = []
hold_xz = []
for i in range(4):
hold_xy.append(board[x][y][i])
hold_yz.append(board[i][y][z])
hold_xz.append(board[x][i][z])
if hold_xy[0] != '_' and are_the_same(hold_xy): return True
if hold_yz[0] != '_' and are_the_same(hold_yz): return True
if hold_xz[0] != '_' and are_the_same(hold_xz): return True
return False
def are_the_same(array):
first = array[0]
for c in array[1:]:
if first != c: return False
return True
def play_OX_3D(moves):
P=({},{})
for counter,(x,y,z) in enumerate(moves,1):
A=(x,x,y,x ,x ,y ,y ,z ,z ,x+y,y+x,z+x,x-y)
B=(y,z,z,y+z,y-z,x+z,x-z,x+y,x-y,x+z,y+z,z+y,y-z)
C=P[counter%2]
for x in zip(range(13),A,B):C[x]=C.get(x,0)+1
if 4 in C.values():return'XO'[counter%2]+' wins after %d moves'%counter
return'No winner'
def play_OX_3DS(moves):
turn = 'O'
counter = 0
board = [[['_' for k in range(4)] for j in range(4)] for i in range(4)]
for move in moves:
x,y,z = move
board[x][y][z] = turn
counter += 1
if check_if_win(board, x, y, z):
return f'{turn} wins after {counter} moves'
if turn == 'O':
turn = 'X'
else:
turn = 'O'
print_board(board)
print(counter)
return 'No winner' | def print_board(board):
for x in board:
for y in x:
for z in y:
print(z,end=' ')
print()
print()
def check_if_win(board, x, y, z):
diags = [
[(0,0,0), (1,1,1), (2,2,2), (3,3,3)],
[(0,0,3), (1,1,2), (2,2,1), (3,3,0)],
[(0,3,0), (1,2,1), (2,1,2), (3,0,3)],
[(0,3,3), (1,2,2), (2,1,1), (3,0,0)],
[(0,0,0), (0,1,1), (0,2,2), (0,3,3)],
[(0,0,3), (0,1,2), (0,2,1), (0,3,0)],
[(1,0,0), (1,1,1), (1,2,2), (1,3,3)],
[(1,0,3), (1,1,2), (1,2,1), (1,3,0)],
[(2,0,0), (2,1,1), (2,2,2), (2,3,3)],
[(2,0,3), (2,1,2), (2,2,1), (2,3,0)],
[(3,0,0), (3,1,1), (3,2,2), (3,3,3)],
[(3,0,3), (3,1,2), (3,2,1), (3,3,0)]
]
for diag in diags:
if (x,y,z) in diag:
d = []
for i,j,k in diag:
d.append(board[i][j][k])
if diag[0] != '_' and are_the_same(d): return True
hold_xy = []
hold_yz = []
hold_xz = []
for i in range(4):
hold_xy.append(board[x][y][i])
hold_yz.append(board[i][y][z])
hold_xz.append(board[x][i][z])
if hold_xy[0] != '_' and are_the_same(hold_xy): return True
if hold_yz[0] != '_' and are_the_same(hold_yz): return True
if hold_xz[0] != '_' and are_the_same(hold_xz): return True
return False
def are_the_same(array):
first = array[0]
for c in array[1:]:
if first != c: return False
return True
def play_OX_3D(moves):
P=({},{})
for counter,(x,y,z) in enumerate(moves,1):
A=(x,x,y,x ,x ,y ,y ,z ,z ,x+y,y+x,z+x,x-y)
B=(y,z,z,y+z,y-z,x+z,x-z,x+y,x-y,x+z,y+z,z+y,y-z)
C=P[counter%2]
for x in zip(range(13),A,B):C[x]=C.get(x,0)+1
if 4 in C.values():return'XO'[counter%2]+' wins after %d moves'%counter
return'No winner'
def play_OX_3DS(moves):
turn = 'O'
counter = 0
board = [[['_' for k in range(4)] for j in range(4)] for i in range(4)]
for move in moves:
x,y,z = move
board[x][y][z] = turn
counter += 1
if check_if_win(board, x, y, z):
return f'{turn} wins after {counter} moves'
if turn == 'O':
turn = 'X'
else:
turn = 'O'
print_board(board)
print(counter)
return 'No winner' | train | APPS_structured |
You are given a sequence of n integers a_1, a_2, ..., a_{n}.
Determine a real number x such that the weakness of the sequence a_1 - x, a_2 - x, ..., a_{n} - x is as small as possible.
The weakness of a sequence is defined as the maximum value of the poorness over all segments (contiguous subsequences) of a sequence.
The poorness of a segment is defined as the absolute value of sum of the elements of segment.
-----Input-----
The first line contains one integer n (1 ≤ n ≤ 200 000), the length of a sequence.
The second line contains n integers a_1, a_2, ..., a_{n} (|a_{i}| ≤ 10 000).
-----Output-----
Output a real number denoting the minimum possible weakness of a_1 - x, a_2 - x, ..., a_{n} - x. Your answer will be considered correct if its relative or absolute error doesn't exceed 10^{ - 6}.
-----Examples-----
Input
3
1 2 3
Output
1.000000000000000
Input
4
1 2 3 4
Output
2.000000000000000
Input
10
1 10 2 9 3 8 4 7 5 6
Output
4.500000000000000
-----Note-----
For the first case, the optimal value of x is 2 so the sequence becomes - 1, 0, 1 and the max poorness occurs at the segment "-1" or segment "1". The poorness value (answer) equals to 1 in this case.
For the second sample the optimal value of x is 2.5 so the sequence becomes - 1.5, - 0.5, 0.5, 1.5 and the max poorness occurs on segment "-1.5 -0.5" or "0.5 1.5". The poorness value (answer) equals to 2 in this case. | import sys
n = int(sys.stdin.readline())
a = [int(x) for x in sys.stdin.readline().split()]
eps = 1e-12
def f(x):
mx = a[0] - x
tsmx = 0.0
mn = a[0] - x
tsmn = 0.0
for ai in a:
tsmx = max(tsmx + ai - x, ai - x)
mx = max(tsmx, mx)
tsmn = min(tsmn + ai - x, ai - x)
mn = min(tsmn, mn)
return abs(mx), abs(mn)
l = min(a)
r = max(a)
f1, f2 = f(l)
for i in range(0, 90):
m = (l + r) / 2
f1, f2 = f(m)
if f1 > f2:
l = m
else:
r = m
A, B = f(l)
print(min(A,B)) | import sys
n = int(sys.stdin.readline())
a = [int(x) for x in sys.stdin.readline().split()]
eps = 1e-12
def f(x):
mx = a[0] - x
tsmx = 0.0
mn = a[0] - x
tsmn = 0.0
for ai in a:
tsmx = max(tsmx + ai - x, ai - x)
mx = max(tsmx, mx)
tsmn = min(tsmn + ai - x, ai - x)
mn = min(tsmn, mn)
return abs(mx), abs(mn)
l = min(a)
r = max(a)
f1, f2 = f(l)
for i in range(0, 90):
m = (l + r) / 2
f1, f2 = f(m)
if f1 > f2:
l = m
else:
r = m
A, B = f(l)
print(min(A,B)) | train | APPS_structured |
A robot is initially at $(0,0)$ on the cartesian plane. It can move in 4 directions - up, down, left, right denoted by letter u, d, l, r respectively. More formally:
- if the position of robot is $(x,y)$ then u makes it $(x,y+1)$
- if the position of robot is $(x,y)$ then l makes it $(x-1,y)$
- if the position of robot is $(x,y)$ then d makes it $(x,y-1)$
- if the position of robot is $(x,y)$ then r makes it $(x+1,y)$
The robot is performing a counter-clockwise spiral movement such that his movement can be represented by the following sequence of moves -
ulddrruuulllddddrrrruuuuu… and so on.
A single move takes 1 sec. You have to find out the position of the robot on the cartesian plane at $t$ second.
-----Input:-----
- The first line of the input contains a single integer $T$ denoting the number of test cases. The description of $T$ test cases follows.
- The first line of each test case contains a single integer $t$.
-----Output:-----
For each test case, print two space-separated integers, $(x,y)$ — the position of the robot.
-----Constraints-----
- $1 \leq T \leq 10^6$
- $1 \leq t \leq 10^{18}$
-----Sample Input:-----
5
1
2
3
50
12233443
-----Sample Output:-----
0 1
-1 1
-1 0
2 4
-1749 812 |
z = int(input())
i = 0
while i < z:
n = int(input())
p = int(n**(0.5))
if p*(p+1) < n:
p += 1
# print("P", p)
x, y = 0, 0
q = 0
flag = True
if p*(p+1) == n:
# print("Even steps, nice")
q = p
else:
# remaining steps
q = p-1
flag = False
if q%2 :
# odd
x -= ((q+1)//2)
y += ((q+1)//2)
else :
x += (q//2)
y -= (q//2)
if flag:
print(x, y)
else:
# remaining steps
l = q*(q+1)
t = p*(p+1)
diff = t-l
# print(x, y)
if x < 0:
# left
if n-l >= diff//2:
y *= (-1)
l += (diff//2)
x += (n-l)
else :
y -= (n-l)
else:
# right
if n-l >= diff//2:
y *= (-1)
y += 1
l += (diff//2)
x -= (n-l)
else :
y += (n-l)
# print("Remaining steps: ", n-l)
print(x, y)
i+=1 |
z = int(input())
i = 0
while i < z:
n = int(input())
p = int(n**(0.5))
if p*(p+1) < n:
p += 1
# print("P", p)
x, y = 0, 0
q = 0
flag = True
if p*(p+1) == n:
# print("Even steps, nice")
q = p
else:
# remaining steps
q = p-1
flag = False
if q%2 :
# odd
x -= ((q+1)//2)
y += ((q+1)//2)
else :
x += (q//2)
y -= (q//2)
if flag:
print(x, y)
else:
# remaining steps
l = q*(q+1)
t = p*(p+1)
diff = t-l
# print(x, y)
if x < 0:
# left
if n-l >= diff//2:
y *= (-1)
l += (diff//2)
x += (n-l)
else :
y -= (n-l)
else:
# right
if n-l >= diff//2:
y *= (-1)
y += 1
l += (diff//2)
x -= (n-l)
else :
y += (n-l)
# print("Remaining steps: ", n-l)
print(x, y)
i+=1 | train | APPS_structured |
Young Sheldon is given the task to teach Chemistry to his brother Georgie. After teaching him how to find total atomic weight, Sheldon gives him some formulas which consist of $x$, $y$ and $z$ atoms as an assignment.
You already know that Georgie doesn't like Chemistry, so he want you to help him solve this assignment.
Let the chemical formula be given by the string $S$. It consists of any combination of x, y and z with some value associated with it as well as parenthesis to encapsulate any combination. Moreover, the atomic weight of x, y and z are 2, 4 and 10 respectively.
You are supposed to find the total atomic weight of the element represented by the given formula.
For example, for the formula $(x_2y_2)_3z$, given string $S$ will be: $(x2y2)3z$. Hence, substituting values of x, y and z, total atomic weight will be
$(2*2+4*2)*3 + 10 = 46$.
-----Input:-----
- First line will contain $T$, number of testcases. Then the testcases follow.
- Each testcase contains of a single line of input $S$.
-----Output:-----
For each testcase, output in a single line, the total atomic weight.
-----Constraints-----
- $1 \leq T \leq 100$
- Length of string $S \leq 100$
- String contains $x, y, z, 1, 2,..., 9$ and parenthesis
-----Sample Input:-----
2
(xy)2
x(x2y)3(z)2
-----Sample Output:-----
12
46 | for _ in range(int(input())):
s = list(input().strip())
i = 0
while i < len(s) - 1:
if s[i].isalpha() or s[i] == ')':
if s[i + 1].isdigit():
if i + 2 >= len(s) or s[i + 2] == ')':
s = s[:i+1] + ['*', s[i+1]] + s[i+2:]
else:
s = s[:i+1] + ['*', s[i+1], '+'] + s[i+2:]
i += 1
elif s[i + 1].isalpha() or s[i + 1] == '(':
s = s[:i+1] + ['+'] + s[i+1:]
i += 1
s = ''.join(s)
s = s.strip('+')
x = 2
y = 4
z = 10
print(eval(s))
| for _ in range(int(input())):
s = list(input().strip())
i = 0
while i < len(s) - 1:
if s[i].isalpha() or s[i] == ')':
if s[i + 1].isdigit():
if i + 2 >= len(s) or s[i + 2] == ')':
s = s[:i+1] + ['*', s[i+1]] + s[i+2:]
else:
s = s[:i+1] + ['*', s[i+1], '+'] + s[i+2:]
i += 1
elif s[i + 1].isalpha() or s[i + 1] == '(':
s = s[:i+1] + ['+'] + s[i+1:]
i += 1
s = ''.join(s)
s = s.strip('+')
x = 2
y = 4
z = 10
print(eval(s))
| train | APPS_structured |
Chef has just found a recipe book, where every dish consists of exactly four ingredients.
He is going to choose some two dishes and prepare them for dinner.
Of course, he likes diversity and wants to know whether the two dishes are similar.
Two dishes are called similar if at least half of their ingredients are the same.
In other words, at least two of four ingredients of the first dish should also be present in the second dish.
The order of ingredients doesn't matter.
Your task is to examine T pairs of dishes.
For each pair, check if the two dishes are similar and print "similar" or "dissimilar" accordingly.
-----Input-----
The first line of the input contains an integer T denoting the number of test cases. The description of T test cases follows.
The first line of each test case contains four distinct strings, denoting ingredients needed for the first dish.
Each ingredient is represented by a string of length between 2 and 10 inclusive, consisting of lowercase English letters.
The second line of each test case describes the second dish in the same format.
-----Output-----
For each test case, output a single line containing the answer — "similar" if at least half of the ingredients are same, and "dissimilar" otherwise (without the quotes).
-----Constraints-----
- 1 ≤ T ≤ 200
- The length of each string will be between 2 and 10 inclusive.
-----Example-----
Input:
5
eggs sugar flour salt
sugar eggs milk flour
aa ab ac ad
ac ad ae af
cookies sugar grass lemon
lemon meat chili wood
one two three four
one two three four
gibberish jibberish lalalalala popopopopo
jibberisz gibberisz popopopopu lalalalalu
Output:
similar
similar
dissimilar
similar
dissimilar
-----Explanation-----
Test case 1. The first dish consists of ingredients: eggs, sugar, flour, salt, while the second dish consists of: sugar, eggs, milk, flour. Three of four ingredients are present in both dishes (eggs, sugar, flour) so the two dishes are similar.
Test case 2. This example shows that strings in the input don't necessarily represent real food.
The answer is again "similar", because two ingredients are present in both dishes ("ac" and "ad").
Test case 3. The only common ingredient is lemon, so the answer is "dissimilar".
Remember that at least two ingredients should be present in both dishes. | n=int(input())
l=[]
for i in range(0,n):
s1=list(map(str,input().split()))
s2=list(map(str,input().split()))
count=0
for j in range(len(s1)):
if(s1[j] in s2):
count+=1
total=len(s1)/2
if(count>=total):
l.append("similar")
else:
l.append("dissimilar")
for i in l:
print(i) | n=int(input())
l=[]
for i in range(0,n):
s1=list(map(str,input().split()))
s2=list(map(str,input().split()))
count=0
for j in range(len(s1)):
if(s1[j] in s2):
count+=1
total=len(s1)/2
if(count>=total):
l.append("similar")
else:
l.append("dissimilar")
for i in l:
print(i) | train | APPS_structured |
Sasha is taking part in a programming competition. In one of the problems she should check if some rooted trees are isomorphic or not. She has never seen this problem before, but, being an experienced participant, she guessed that she should match trees to some sequences and then compare these sequences instead of trees. Sasha wants to match each tree with a sequence a_0, a_1, ..., a_{h}, where h is the height of the tree, and a_{i} equals to the number of vertices that are at distance of i edges from root.
Unfortunately, this time Sasha's intuition was wrong, and there could be several trees matching the same sequence. To show it, you need to write a program that, given the sequence a_{i}, builds two non-isomorphic rooted trees that match that sequence, or determines that there is only one such tree.
Two rooted trees are isomorphic, if you can reenumerate the vertices of the first one in such a way, that the index of the root becomes equal the index of the root of the second tree, and these two trees become equal.
The height of a rooted tree is the maximum number of edges on a path from the root to any other vertex.
-----Input-----
The first line contains a single integer h (2 ≤ h ≤ 10^5) — the height of the tree.
The second line contains h + 1 integers — the sequence a_0, a_1, ..., a_{h} (1 ≤ a_{i} ≤ 2·10^5). The sum of all a_{i} does not exceed 2·10^5. It is guaranteed that there is at least one tree matching this sequence.
-----Output-----
If there is only one tree matching this sequence, print "perfect".
Otherwise print "ambiguous" in the first line. In the second and in the third line print descriptions of two trees in the following format: in one line print $\sum_{i = 0}^{h} a_{i}$ integers, the k-th of them should be the parent of vertex k or be equal to zero, if the k-th vertex is the root.
These treese should be non-isomorphic and should match the given sequence.
-----Examples-----
Input
2
1 1 1
Output
perfect
Input
2
1 2 2
Output
ambiguous
0 1 1 3 3
0 1 1 3 2
-----Note-----
The only tree in the first example and the two printed trees from the second example are shown on the picture:
$88$ | h = int(input())
a = list(map(int, input().split()))
w, q = [], []
p = r = 0
for i in a:
for j in range(i):
w.append(r)
q.append(r - (j and p > 1))
p = i
r += i
if w == q:
print('perfect')
else:
print('ambiguous')
print(*w)
print(*q)
| h = int(input())
a = list(map(int, input().split()))
w, q = [], []
p = r = 0
for i in a:
for j in range(i):
w.append(r)
q.append(r - (j and p > 1))
p = i
r += i
if w == q:
print('perfect')
else:
print('ambiguous')
print(*w)
print(*q)
| train | APPS_structured |
You're writing an excruciatingly detailed alternate history novel set in a world where [Daniel Gabriel Fahrenheit](https://en.wikipedia.org/wiki/Daniel_Gabriel_Fahrenheit) was never born.
Since Fahrenheit never lived the world kept on using the [Rømer scale](https://en.wikipedia.org/wiki/R%C3%B8mer_scale), invented by fellow Dane [Ole Rømer](https://en.wikipedia.org/wiki/Ole_R%C3%B8mer) to this very day, skipping over the Fahrenheit and Celsius scales entirely.
Your magnum opus contains several thousand references to temperature, but those temperatures are all currently in degrees Celsius. You don't want to convert everything by hand, so you've decided to write a function, `celsius_to_romer()` that takes a temperature in degrees Celsius and returns the equivalent temperature in degrees Rømer.
For example: `celsius_to_romer(24)` should return `20.1`. | celsius_to_romer=lambda x:(x*21.0/40)+7.5 | celsius_to_romer=lambda x:(x*21.0/40)+7.5 | train | APPS_structured |
Ahmed Gafer failed to pass the test, but he got the job because of his friendship with Said and Shahhoud. After working in the kitchen for a while, he blew it. The customers didn't like the food anymore and one day he even burned the kitchen. Now the master Chef is very upset.
Ahmed isn't useful anymore at being a co-Chef, so S&S decided to give him a last chance. They decided to give Ahmed a new job, and make him work as the cashier of the restaurant. Nevertheless, in order not to repeat their previous mistake, they decided to give him a little test to check if his counting skills are good enough for the job. The problem is as follows:
Given a string A of lowercase English letters, Ahmad was asked to find the number of good substrings.
A substring A[L, R] is good if:
- The length of the substring is exactly 2 and AL = AR, OR
- The length of the substring is greater than 2,AL = AR and the substring A[L + 1, R - 1] has only one distinct letter.
Anyways, Ahmed struggled trying to find a solution for the problem. Since his mathematical skills are very poor as it turned out, he decided to cheat and contacted you asking for your help. Can you help him in this challenge?
-----Input-----
The first line of the input contains the integer T, indicating the number of test cases.
Each of the following T lines, contains a string A.
-----Output-----
For each test case, output a single line containing a single number, indicating the number of good substrings.
-----Constraints-----
- 1 ≤ T ≤ 100
- 1 ≤ |A| ≤ 105
- It's guaranteed that the sum of |A| over all test cases doesn't exceed 5x105.
-----Example-----
Input:
2
a
abba
Output:
0
2
-----Explanation-----
Example case 2. The good substrings of abba are: { bb } and { abba }. | # cook your dish here
for _ in range(int(input())):
s=input()
count=0
i=0
while i<len(s)-1:
ch=s[i]
j=i+1
while j<len(s) and s[j]==ch:
j+=1
l=j-i
if i!=0 and j!=len(s) and s[i-1]==s[j] :
count+=1
count+=l*(l-1)//2
#print(s[i:j],count)
i=j
print(count)
| # cook your dish here
for _ in range(int(input())):
s=input()
count=0
i=0
while i<len(s)-1:
ch=s[i]
j=i+1
while j<len(s) and s[j]==ch:
j+=1
l=j-i
if i!=0 and j!=len(s) and s[i-1]==s[j] :
count+=1
count+=l*(l-1)//2
#print(s[i:j],count)
i=j
print(count)
| train | APPS_structured |
For "x", determine how many positive integers less than or equal to "x" are odd but not prime. Assume "x" is an integer between 1 and 10000.
Example: 5 has three odd numbers (1,3,5) and only the number 1 is not prime, so the answer is 1
Example: 10 has five odd numbers (1,3,5,7,9) and only 1 and 9 are not prime, so the answer is 2 | def odd_not_prime(n):
return len([x for x in range(1, n+1, 2) if not (not any([x%y==0 for y in range(3, int(x**(1/2))+1, 2)]) and x!=1)]) | def odd_not_prime(n):
return len([x for x in range(1, n+1, 2) if not (not any([x%y==0 for y in range(3, int(x**(1/2))+1, 2)]) and x!=1)]) | train | APPS_structured |
Bob has n heap(s) of gravel (initially there are exactly c piece(s) in each). He wants to do m operation(s) with that heaps, each maybe:
- adding pieces of gravel onto the heaps from u to v, exactly k pieces for each,
- or querying "how many pieces of gravel are there in the heap p now?".
-----Request-----
Help Bob do operations of the second type.
-----Input-----
- The first line contains the integers n,m,c, respectively.
- m following lines, each forms:
- S u v k to describe an operation of the first type.
- Q p to describe an operation of the second type.
(Each integer on a same line, or between the characters S, Q and the integers is separated by at least one space character)
-----Output-----
For each operation of the second type, output (on a single line) an integer answering to the respective query (follows the respective Input order).
-----Example-----Input:
7 5 0
Q 7
S 1 7 1
Q 3
S 1 3 1
Q 3
Output:
0
1
2
-----Limitations-----
- 0<n≤106
- 0<m≤250 000
- 0<u≤v≤n
- 0≤c,k≤109
- 0<p≤n | def update(fenwick,n,ind,val):
while(ind<=n):
fenwick[ind]+=val;
ind+=ind&(-ind)
def get(arr,ind):
su=0
while(ind>0):
su+=arr[ind]
ind-=ind&(-ind)
return su
n,m,c=list(map(int,input().strip().split(" ")))
fenwick=[0]*(n+1)
for z in range(m):
s=list(map(str,input().strip().split(" ")))
if(s[0]=='S'):
update(fenwick,n,(int)(s[1]),(int)(s[3]))
update(fenwick,n,(int)(s[2])+1,-(int)(s[3]))
else:
print(get(fenwick,(int)(s[1]))+c)
| def update(fenwick,n,ind,val):
while(ind<=n):
fenwick[ind]+=val;
ind+=ind&(-ind)
def get(arr,ind):
su=0
while(ind>0):
su+=arr[ind]
ind-=ind&(-ind)
return su
n,m,c=list(map(int,input().strip().split(" ")))
fenwick=[0]*(n+1)
for z in range(m):
s=list(map(str,input().strip().split(" ")))
if(s[0]=='S'):
update(fenwick,n,(int)(s[1]),(int)(s[3]))
update(fenwick,n,(int)(s[2])+1,-(int)(s[3]))
else:
print(get(fenwick,(int)(s[1]))+c)
| train | APPS_structured |
## Task
Given a positive integer, `n`, return the number of possible ways such that `k` positive integers multiply to `n`. Order matters.
**Examples**
```
n = 24
k = 2
(1, 24), (2, 12), (3, 8), (4, 6), (6, 4), (8, 3), (12, 2), (24, 1) -> 8
n = 100
k = 1
100 -> 1
n = 20
k = 3
(1, 1, 20), (1, 2, 10), (1, 4, 5), (1, 5, 4), (1, 10, 2), (1, 20, 1),
(2, 1, 10), (2, 2, 5), (2, 5, 2), (2, 10, 1), (4, 1, 5), (4, 5, 1),
(5, 1, 4), (5, 2, 2), (5, 4, 1), (10, 1, 2), (10, 2, 1), (20, 1, 1) -> 18
```
**Constraints**
`1 <= n <= 500_000_000`
and `1 <= k <= 1000` | from math import sqrt,factorial
def c(n,m):
return factorial(n)//(factorial(n-m)*factorial(m))
def factor(n):#Amount of prime numbers
factors={}
max=int(sqrt(n)+1)
f=2
while f<=max:
if n%f==0:
factors[f]=0
while n%f==0:
factors[f]+=1
n//=f
max=int(sqrt(n)+1)
f+=1
if n!=1:
factors[n]=1
return factors
def multiply(n, k):
mul=1
for m in factor(n).values():
mul*=c(m+k-1,k-1)
return mul | from math import sqrt,factorial
def c(n,m):
return factorial(n)//(factorial(n-m)*factorial(m))
def factor(n):#Amount of prime numbers
factors={}
max=int(sqrt(n)+1)
f=2
while f<=max:
if n%f==0:
factors[f]=0
while n%f==0:
factors[f]+=1
n//=f
max=int(sqrt(n)+1)
f+=1
if n!=1:
factors[n]=1
return factors
def multiply(n, k):
mul=1
for m in factor(n).values():
mul*=c(m+k-1,k-1)
return mul | train | APPS_structured |
Mrs Jefferson is a great teacher. One of her strategies that helped her to reach astonishing results in the learning process is to have some fun with her students. At school, she wants to make an arrangement of her class to play a certain game with her pupils. For that, she needs to create the arrangement with **the minimum amount of groups that have consecutive sizes**.
Let's see. She has ```14``` students. After trying a bit she could do the needed arrangement:
```[5, 4, 3, 2]```
- one group of ```5``` students
- another group of ```4``` students
- then, another one of ```3```
- and finally, the smallest group of ```2``` students.
As the game was a success, she was asked to help to the other classes to teach and show the game. That's why she desperately needs some help to make this required arrangements that make her spend a lot of time.
To make things worse, she found out that there are some classes with some special number of students that is impossible to get that arrangement.
Please, help this teacher!
Your code will receive the number of students of the class. It should output the arrangement as an array with the consecutive sizes of the groups in decreasing order.
For the special case that no arrangement of the required feature is possible the code should output ```[-1] ```
The value of n is unknown and may be pretty high because some classes joined to to have fun with the game.
You may see more example tests in the Example Tests Cases Box. | def shortest_arrang(n):
# For odd n, we can always construct n with 2 consecutive integers.
if n % 2 == 1:
return [n//2 + 1, n//2]
# For even n, n is the sum of either an odd number or even number of
# consecutive positive integers. Moreover, this property is exclusive.
for i in range(3, n // 2):
if i % 2 == 1 and n % i == 0:
# For odd i, if n / i is an integer, then the sequence, which has
# odd length, is centered around n / i.
return list(range(n//i + i//2, n//i - i//2 - 1, -1))
elif i % 2 == 0 and n % i == i // 2:
# For even i, if the remainder of n / i is 1/2, then the sequence
# (even length) is centered around n / i.
return list(range(n//i + i//2, n//i - i//2, -1))
# If none of the above are satisfied, then n is a power of 2 and we cannot
# write it as the sum of two consecutive integers.
return [-1]
| def shortest_arrang(n):
# For odd n, we can always construct n with 2 consecutive integers.
if n % 2 == 1:
return [n//2 + 1, n//2]
# For even n, n is the sum of either an odd number or even number of
# consecutive positive integers. Moreover, this property is exclusive.
for i in range(3, n // 2):
if i % 2 == 1 and n % i == 0:
# For odd i, if n / i is an integer, then the sequence, which has
# odd length, is centered around n / i.
return list(range(n//i + i//2, n//i - i//2 - 1, -1))
elif i % 2 == 0 and n % i == i // 2:
# For even i, if the remainder of n / i is 1/2, then the sequence
# (even length) is centered around n / i.
return list(range(n//i + i//2, n//i - i//2, -1))
# If none of the above are satisfied, then n is a power of 2 and we cannot
# write it as the sum of two consecutive integers.
return [-1]
| train | APPS_structured |
You need to write a function that reverses the words in a given string. A word can also fit an empty string. If this is not clear enough, here are some examples:
As the input may have trailing spaces, you will also need to ignore unneccesary whitespace.
```python
reverse('Hello World') == 'World Hello'
reverse('Hi There.') == 'There. Hi'
```
Happy coding! | def reverse(st):
x = st.split()
x = list(reversed(x))
return (" ".join(x)) | def reverse(st):
x = st.split()
x = list(reversed(x))
return (" ".join(x)) | train | APPS_structured |
Given a square matrix (i.e. an array of subarrays), find the sum of values from the first value of the first array, the second value of the second array, the third value of the third array, and so on...
## Examples
```
array = [[1, 2],
[3, 4]]
diagonal sum: 1 + 4 = 5
```
```
array = [[5, 9, 1, 0],
[8, 7, 2, 3],
[1, 4, 1, 9],
[2, 3, 8, 2]]
diagonal sum: 5 + 7 + 1 + 2 = 15
``` | def diagonal_sum(a):
return sum(l[i] for i, l in enumerate(a)) | def diagonal_sum(a):
return sum(l[i] for i, l in enumerate(a)) | train | APPS_structured |
Subsets and Splits