markdown
stringlengths 0
1.02M
| code
stringlengths 0
832k
| output
stringlengths 0
1.02M
| license
stringlengths 3
36
| path
stringlengths 6
265
| repo_name
stringlengths 6
127
|
---|---|---|---|---|---|
Skeletonize | import numpy as np
import cv2
from imutils import resize
from imutils.contours import sort_contours
from skimage.morphology import skeletonize as skl
# path = 'test_img/cat.png'
# path = "test_img/place.png"
# path = "test_img/cts.png"
path = "test_img/"+image_name
# path = 'test_img/reverse.png'
img = cv2.imread(path, 0)
# Some smoothing to get rid of the noise
# img = cv2.bilateralFilter(img, 5, 35, 10)
img = cv2.GaussianBlur(img, (3, 3), 3)
# img = resize(img, width=700)
# Preprocessing to get the shapes
th = cv2.adaptiveThreshold(img, 255, cv2.ADAPTIVE_THRESH_GAUSSIAN_C,
cv2.THRESH_BINARY, 35, 11)
# Invert to hightligth the shape
th = cv2.bitwise_not(th)
# Text has mostly vertical and right-inclined lines. This kernel seems to
# work quite well
kernel = np.array([[0, 1, 1],
[0, 1, 0],
[1, 1, 0]], dtype='uint8')
th = cv2.morphologyEx(th, cv2.MORPH_CLOSE, kernel)
cv2.imshow('mask', th)
cv2.waitKey(0)
#def contour_sorter(contours):
# '''Sort the contours by multiplying the y-coordinate and sorting first by
# x, then by y-coordinate.'''
# boxes = [cv2.boundingRect(c) for c in contours]
# cnt = [4*y, x for y, x, , _, _ in ]
# Skeletonize the shapes
# Skimage function takes image with either True, False or 0,1
# and returns and image with values 0, 1.
th = th == 255
th = skl(th)
th = th.astype(np.uint8)*255
# Find contours of the skeletons
_, contours, _ = cv2.findContours(th.copy(), cv2.RETR_EXTERNAL,
cv2.CHAIN_APPROX_NONE)
# Sort the contours left-to-rigth
contours, _ = sort_contours(contours, )
#
# Sort them again top-to-bottom
def skeleton_endpoints(skel):
# Function source: https://stackoverflow.com/questions/26537313/
# how-can-i-find-endpoints-of-binary-skeleton-image-in-opencv
# make out input nice, possibly necessary
skel = skel.copy()
skel[skel != 0] = 1
skel = np.uint8(skel)
# apply the convolution
kernel = np.uint8([[1, 1, 1],
[1, 10, 1],
[1, 1, 1]])
src_depth = -1
filtered = cv2.filter2D(skel, src_depth,kernel)
# now look through to find the value of 11
# this returns a mask of the endpoints, but if you just want the
# coordinates, you could simply return np.where(filtered==11)
out = np.zeros_like(skel)
out[np.where(filtered == 11)] = 1
rows, cols = np.where(filtered == 11)
coords = list(zip(cols, rows))
return coords
# List for endpoints
endpoints = []
# List for (x, y) coordinates of the skeletons
skeletons = []
for contour in contours:
if cv2.arcLength(contour, True) > 100:
# Initialize mask
mask = np.zeros(img.shape, np.uint8)
# Bounding rect of the contour
x, y, w, h = cv2.boundingRect(contour)
mask[y:y+h, x:x+w] = 255
# Get only the skeleton in the mask area
mask = cv2.bitwise_and(mask, th)
# Take the coordinates of the skeleton points
rows, cols = np.where(mask == 255)
# Add the coordinates to the list
skeletons.append(list(zip(cols, rows)))
# Find the endpoints for the shape and update a list
eps = skeleton_endpoints(mask)
endpoints.append(eps)
# Draw the endpoints
# [cv2.circle(th, ep, 5, 255, 1) for ep in eps]
cv2.imshow('mask', mask)
cv2.waitKey(500)
# cv2.imwrite("res/l_cat.png",th)# Stack the original and modified
# cv2.imwrite("res/l_reverse.png",th)# Stack the original and modified
# cv2.imwrite("res/l_place.png",th)# Stack the original and modified
# cv2.imwrite("res/l_cts.png",th)# Stack the original and modified
cv2.imwrite("res/l_"+image_name+".png",th)# Stack the original and modified
th = resize(np.hstack((img, th)), 1200)
# cv2.waitKey(50)
# TODO
# Walk the points using the endpoints by minimizing the walked distance
# Points in between can be used many times, endpoints only once
cv2.imshow('mask', th)
cv2.waitKey(0)
cv2.destroyAllWindows()
# skel_img = cv2.imread("res/l_cat.png",0)
# skel_img = cv2.imread("res/l_place.png",0)
# skel_img = cv2.imread("res/l_cts.png",0)
skel_img = cv2.imread("res/l_"+image_name+".png",0)
# skel_img = cv2.imread("res/l_reverse.png",0)
plt.imshow(skel_img) | _____no_output_____ | MIT | Notebooks/.ipynb_checkpoints/Character Segmentation Model -checkpoint.ipynb | swapnilmarathe007/Handwriting-Recognition |
Turn to transpose | trnspse_img = np.transpose(skel_img)
plt.imshow(trnspse_img) | _____no_output_____ | MIT | Notebooks/.ipynb_checkpoints/Character Segmentation Model -checkpoint.ipynb | swapnilmarathe007/Handwriting-Recognition |
Calculate the median of the word | up = []
down = []
for val in trnspse_img:
temp = []
for i , value in enumerate(val):
if(value>0):
temp.append(i)
try:
up.append(temp[0])
down.append(temp[-1])
except :
pass
up_avg = sum(up)//len(up)
print (up_avg)
down_avg = sum(down) // len(down)
print (down_avg)
median = ( up_avg + down_avg ) // 2
print (median)
copy_of_original = skel_img.copy()
plt.imshow(copy_of_original) | _____no_output_____ | MIT | Notebooks/.ipynb_checkpoints/Character Segmentation Model -checkpoint.ipynb | swapnilmarathe007/Handwriting-Recognition |
Draw line in median in copy | copy_of_original[median] = [255] * len(copy_of_original[median])
plt.imshow(copy_of_original)
# Transpose look of median drawn image
plt.imshow(np.transpose(copy_of_original))
# checking from down till median for single 255 and sum of that val == 255
sp_list = [] # Segmentation Points
for i , val in enumerate(trnspse_img):
if (sum(val[median:]) == 255 and sum(val) == 255):
sp_list.append(i)
#from Top to bottom
# checking from down till median for single 255 and sum of that val == 255
sp_list_t = [] # Segmentation Points
for i , val in enumerate(trnspse_img):
if (sum(val[:median]) == 255 and sum(val) == 255):
sp_list_t.append(i)
# print(sp_list_t)
print (sp_list) | [193, 194, 195, 196, 197, 198, 199, 200, 201, 202, 203, 204, 205, 206, 249, 250, 251, 252, 253, 254, 255, 256, 257, 258, 259, 260, 261, 262, 263, 264, 265, 266, 267, 268, 269, 270, 271, 272, 274, 276, 311, 312, 313, 314, 315, 316, 317, 318, 319, 320, 321, 322, 323, 324, 325, 326, 327, 328, 329, 330, 384, 385, 386, 387, 388, 389, 390, 391, 392, 393, 394, 395, 396, 397, 398, 399, 402, 403, 419, 421, 425, 426, 427, 428, 429, 431, 433, 434, 463, 464, 465, 466, 467, 468, 469, 470, 471, 472, 473, 474, 475, 476, 477, 478, 479, 480, 481, 482, 483, 484, 485, 486, 487, 488, 489, 490, 491, 492, 493, 494, 495, 496, 497, 498, 499, 500, 501, 502, 503, 504, 505, 506, 507, 508, 509, 510, 511, 512, 513, 514, 515, 516, 517, 518, 519, 520, 521, 522, 523, 524, 525, 526, 527, 528, 529, 530]
| MIT | Notebooks/.ipynb_checkpoints/Character Segmentation Model -checkpoint.ipynb | swapnilmarathe007/Handwriting-Recognition |
To find Consecutive elements | def consecutive(data, stepsize=1):
return np.split(data, np.where(np.diff(data) != stepsize)[0]+1)
res_list = consecutive(sp_list)
# from Top
res_list_t = consecutive(sp_list_t)
for lst in res_list:
print (lst)
#from Top
for lst in res_list_t:
print (lst)
avg_of_blocks = []
#from top
avg_of_blocks_t = []
for lst in res_list:
# if(len(lst) > 7):
avg_of_blocks.append(sum(lst)//len(lst))
print(avg_of_blocks_t)
#from Top
for lst in res_list_t:
# if(len(lst) > ):
avg_of_blocks_t.append(sum(lst)//len(lst))
# Saving splliting of u , v, w
new_avg_block = []
new_avg_block.append(avg_of_blocks[0])
for i , val in enumerate(avg_of_blocks):
try:
temp = (avg_of_blocks[i+1]-avg_of_blocks[i])
if(temp > 60):
new_avg_block.append(avg_of_blocks[i+1])
except:
pass
#from Top
# Saving splliting of m , n , h ,
new_avg_block_t = []
new_avg_block_t.append(avg_of_blocks_t[0])
for i , val in enumerate(avg_of_blocks_t):
try:
temp = (avg_of_blocks_t[i+1]-avg_of_blocks_t[i])
if(temp > 60):
new_avg_block_t.append(avg_of_blocks_t[i+1])
except:
pass
print (new_avg_block)
print (new_avg_block_t) | [85, 279, 353]
| MIT | Notebooks/.ipynb_checkpoints/Character Segmentation Model -checkpoint.ipynb | swapnilmarathe007/Handwriting-Recognition |
This is a new way of hacking | combine_copy = skel_img.copy()
plt.imshow(combine_copy)
new_combined_list = [] + new_avg_block
for val in new_avg_block_t:
for i , vl in enumerate(new_avg_block):
try:
if(val-vl > 80 and new_avg_block[i+1]-val > 80):
new_combined_list.append(val)
break
except:
pass
new_combined_list = sorted(new_combined_list)
new_transpose_copy = np.transpose(combine_copy)
plt.imshow(new_transpose_copy)
for val in new_combined_list:
new_transpose_copy[val] = [255] * len(new_transpose_copy[val])
plt.imshow(new_transpose_copy)
# cv2.imwrite("res/res_cts_combined.png",np.transpose(new_transpose_copy))
cv2.imwrite("res/res_"+image_name+"_combined.png",np.transpose(new_transpose_copy))
# cv2.imwrite("res/res_place_combined.png",np.transpose(new_transpose_copy)) | _____no_output_____ | MIT | Notebooks/.ipynb_checkpoints/Character Segmentation Model -checkpoint.ipynb | swapnilmarathe007/Handwriting-Recognition |
Tried but not working | transpose_of_copy = np.transpose(copy_of_original)
#for top
transpose_of_copy_t = np.transpose(copy_of_original)
plt.imshow(transpose_of_copy)
plt.imshow(transpose_of_copy_t)
# for val in avg_of_blocks:
# transpose_of_copy[val] = [255] * len(transpose_of_copy[val])
for val in new_avg_block:
transpose_of_copy[val] = [255] * len(transpose_of_copy[val])
#from Top
# for val in avg_of_blocks:
# transpose_of_copy[val] = [255] * len(transpose_of_copy[val])
for val in new_avg_block_t:
transpose_of_copy_t[val] = [255] * len(transpose_of_copy_t[val])
plt.imshow(transpose_of_copy)
plt.imshow(transpose_of_copy_t)
plt.imshow(np.transpose(transpose_of_copy))
plt.imshow(np.transpose(transpose_of_copy_t))
# cv2.imwrite("res/res_reverse.png",np.transpose(transpose_of_copy))
# cv2.imwrite("res/res_place.png",np.transpose(transpose_of_copy))
# cv2.imwrite("res/res_cts.png",np.transpose(transpose_of_copy))
cv2.imwrite("res/res_"+image_name+".png",np.transpose(transpose_of_copy))
#from Top
# cv2.imwrite("res/res_reverse.png",np.transpose(transpose_of_copy))
# cv2.imwrite("res/res_place.png",np.transpose(transpose_of_copy))
cv2.imwrite("res/res_"+image_name+"_t.png",np.transpose(transpose_of_copy_t))
# Checking for a way to save u, v, w
print (avg_of_blocks)
print (new_avg_block)
#checking diff between next and this
for i , val in enumerate(avg_of_blocks):
try:
print (avg_of_blocks[i+1]-avg_of_blocks[i])
except:
pass
#checking diff between next and this
for i , val in enumerate(new_avg_block):
try:
print (new_avg_block[i+1]-new_avg_block[i])
except:
pass
| 61
131
105
| MIT | Notebooks/.ipynb_checkpoints/Character Segmentation Model -checkpoint.ipynb | swapnilmarathe007/Handwriting-Recognition |
两种降维的途径:投影$(projection)$和流形学习$(Manifold\ Learning)$ 三种降维的技术:$PCA, Kernel\ PCA, LLE$ | # 2D
import numpy as np
p = 0
for it in range(100001):
x1, y1 = np.random.ranf(), np.random.ranf()
x2, y2 = np.random.ranf(), np.random.ranf()
p += np.sqrt((x1 - x2) ** 2 + (y1 - y2) ** 2)
print(1.0 * p / 100000)
# 3D
p = 0
for it in range(100001):
x1, y1, z1 = np.random.ranf(), np.random.ranf(), np.random.ranf()
x2, y2, z2 = np.random.ranf(), np.random.ranf(), np.random.ranf()
p += np.sqrt((x1 - x2) ** 2 + (y1 - y2) ** 2 + (z1-z2) ** 2)
print(1.0 * p / 100000)
# 7D
p = 0
for it in range(100001):
(x1, y1, z1, a1, b1, c1, d1, e1) = (np.random.ranf(), np.random.ranf(), np.random.ranf(), np.random.ranf(),
np.random.ranf(), np.random.ranf(), np.random.ranf(), np.random.ranf())
(x2, y2, z2, a2, b2, c2, d2, e2) = (np.random.ranf(), np.random.ranf(), np.random.ranf(), np.random.ranf(),
np.random.ranf(), np.random.ranf(), np.random.ranf(), np.random.ranf())
p += np.sqrt((x1-x2)**2+(y1-y2)**2+(z1-z2)**2+(a1-a2)**2 +(b1-b2)**2+(c1-c2)**2+(d1-d2)**2+(e1-e2)**2)
print(1.0 * p / 100000) | 1.1286641926694834
| MIT | handson-ml/8_Dimensionality Reduction.ipynb | Luoyayu/Machine-Learning |
由上述计算可知,当维数增加时,hypercube中两点距离变大,当维数为1e6时,avg_dis=408.25,可知在高维空间内数据点间隔较大,分布非常稀疏, 这意味着遇到的new instace 也可能距离所有的train instances很远, 从而导致预测相比低维空间不可靠, 通常表现为overfitting, 因为模型做了很强的外推。一种直观的解决方法是增大数据密度然而显然这是不切实际的。 Main Approaches for Dimensionality Reduction Projection 投影法投影法基于这样的事实:虽然数据是多维度的,但是数据之间强关联性或者某类特征为常量,这样产生的数据集就很有可能仅仅lie with很低的维度,比如下面的3D数据 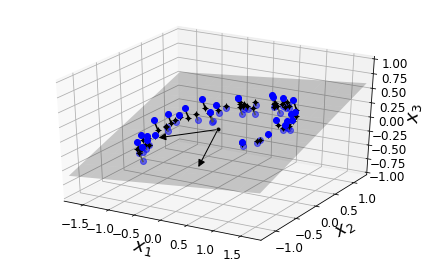 可以看出数据仅在灰色平面上, 因此我们可以做这样的降维处理 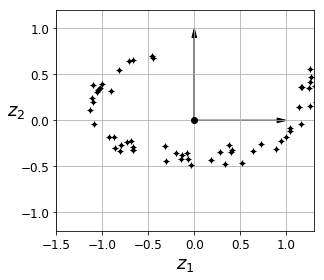 但是不是所有的数据集合都是可以简单通过投影进行降维的,对于Swiss roll dataset,我们希望能有更好的方法降低维度 Swiss rollm | from sklearn.datasets import make_swiss_roll
import matplotlib.pylab as plt
from mpl_toolkits.mplot3d import Axes3D
X, t = make_swiss_roll(n_samples=1000, noise=0.2, random_state=42)
axes = [-11.5, 14, -2, 23, -12, 15]
fig = plt.figure(figsize=(10, 10))
ax = fig.add_subplot(111, projection='3d')
ax.scatter(X[:, 0], X[:, 1], X[:, 2], c=t, cmap=plt.cm.hot) # 绘点
ax.view_init(10, -70) # 视角
ax.set_xlim(axes[0:2])
ax.set_ylim(axes[2:4])
ax.set_zlim(axes[4:6])
plt.show() | _____no_output_____ | MIT | handson-ml/8_Dimensionality Reduction.ipynb | Luoyayu/Machine-Learning |
我们应该把瑞士卷拉开展平在2D上,而不是直接拍平 | plt.figure(figsize=(18, 6))
plt.subplot(121)
plt.scatter(X[:, 0], X[:, 1], c=t, cmap=plt.cm.hot)
plt.axis('off')
plt.subplot(122)
plt.scatter(t, X[:,1], c=t, cmap=plt.cm.hot)
plt.axis('off')
plt.show() | _____no_output_____ | MIT | handson-ml/8_Dimensionality Reduction.ipynb | Luoyayu/Machine-Learning |
Manifold Learning 所谓流形是指d维的超平面在更高的n维空间被bent,twist, 比如上面的右图其在作为2D平面在3D空间被roll后形成了3D瑞士卷 PCA Principal Component Analysis | np.random.seed(4)
m = 60
w1, w2 = 0.1, 0.3
noise = 0.1
angles = np.random.rand(m) * 3 * np.pi / 2 - 0.5
X = np.empty((m, 3))
X[:, 0] = np.cos(angles) + np.sin(angles)/2 + noise * np.random.randn(m) / 2
X[:, 1] = np.sin(angles) * 0.7 + noise * np.random.randn(m) / 2
X[:, 2] = X[:, 0] * w1 + X[:, 1] * w2 + noise * np.random.randn(m) | _____no_output_____ | MIT | handson-ml/8_Dimensionality Reduction.ipynb | Luoyayu/Machine-Learning |
Principal Component(PCs) | # 使用SVD(奇异值分解)求主成分PCs
import numpy as np
X_centered = X - X.mean(axis=0)
U, s, V = np.linalg.svd(X_centered)
c1 = V[:, 0]
c2 = V[:, 1]
c1, c2 # PCs
c1.dot(c2) # 正交
W2 = V.T[:, :2]
X2D = X_centered.dot(W2)
from sklearn.decomposition import PCA
pca = PCA(n_components = 2)
X2D = pca.fit_transform(X) # automatic centering
print(pca.explained_variance_ratio_) # 可解释方差比, 反映了特征所在轴的方差占比 | [0.84248607 0.14631839]
| MIT | handson-ml/8_Dimensionality Reduction.ipynb | Luoyayu/Machine-Learning |
Choose the Right Number of Dimensions | # 假设我们要求降维后的数据保存95%的信息
pca = PCA()
pca.fit(X)
cumsum = np.cumsum(pca.explained_variance_ratio_) # 前缀和cumsum
d = np.argmax(cumsum >= 0.95) + 1
print(d, cumsum[d-1])
pca = PCA(n_components=d) # or n_components = 0.95 | 2 0.988804464429311
| MIT | handson-ml/8_Dimensionality Reduction.ipynb | Luoyayu/Machine-Learning |
PCA for Compression | from sklearn.datasets import fetch_mldata
from sklearn.model_selection import train_test_split
import matplotlib
mnist = fetch_mldata("mnist original")
X = mnist['data']
y = mnist['target']
X_train, X_test, y_train, y_test = train_test_split(X, y)
def plot_digit(flat, size=28):
img = flat.reshape(size, size)
plt.imshow(img, cmap=matplotlib.cm.binary, interpolation='nearest')
s = 5555
some_digit = X_train[s]
plt.figure(figsize=(12,6))
plt.subplot(221)
plt.title('number <{}>'.format(int(y[s])))
plot_digit(some_digit)
plt.subplot(222)
pca = PCA(n_components=169)
X_reduced = pca.fit_transform(X_train)
X_recoverd = pca.inverse_transform(X_reduced)
plot_digit(X_recoverd[s]) # 绘制解压图像
plt.show() | _____no_output_____ | MIT | handson-ml/8_Dimensionality Reduction.ipynb | Luoyayu/Machine-Learning |
Incremental PCA | %matplotlib notebook
from sklearn.decomposition import IncrementalPCA
import numpy as np
n_batchs = 100
inc_pac = path = IncrementalPCA(n_components=169)
for X_batch in np.array_split(X_train, n_batchs):
inc_pac.partial_fit(X_batch)
X_mnist_reduced = inc_pac.fit_transform(X_train)
X_mnist_recoverd = inc_pac.inverse_transform(X_mnist_reduced)
plot_digit(X_mnist_recoverd[s])
import cv2 as cv
img = cv.imread('/Users/hu-osx/Desktop/ER.jpg')
img = cv.cvtColor(img, cv.COLOR_BGR2RGB)
plt.imshow(img)
plt.show() | _____no_output_____ | MIT | handson-ml/8_Dimensionality Reduction.ipynb | Luoyayu/Machine-Learning |
[Module 1.2] 세이지 메이커 로컬 모드 및 스크립트 모드로 훈련본 워크샵의 모든 노트북은 **conda_tensorflow2_p36** 를 사용합니다.이 노트북은 아래와 같은 작업을 합니다.- 1. 기본 환경 세팅 - 2. 노트북에서 세이지 메이커 스크립트 모드 스타일로 코드 변경- 3. 세이지 메이커 스크립트 모드 스타일로 코드 실행 (실제로 세이지 메이커 사용 안함)- 4. 세이지 메이커 로컬 모드로 훈련- 5. 세이지 메이커의 호스트 모드로 훈련- 6. 모델 아티펙트 경로 저장 참고:- 이 페이지를 보시면 Cifar10 데이터 설명 및 기본 모델 훈련이 있습니다. --> [Train a Keras Sequential Model (TensorFlow 2.0)](https://github.com/daekeun-ml/tensorflow-in-sagemaker-workshop/blob/master/0_Running_TensorFlow_In_SageMaker_tf2.ipynb) - 메인 깃 리파지토리: [SageMaker Workshop: Tensorflow-Keras 모델을 Amazon SageMaker에서 학습하기](https://github.com/daekeun-ml/tensorflow-in-sagemaker-workshop)--- 1. 기본 환경 세팅사용하는 패키지는 import 시점에 다시 재로딩 합니다. | %load_ext autoreload
%autoreload 2
import sagemaker
sagemaker_session = sagemaker.Session()
bucket = sagemaker_session.default_bucket()
prefix = "sagemaker/DEMO-pytorch-cnn-cifar10"
role = sagemaker.get_execution_role()
import tensorflow as tf
print("tensorflow version: ", tf.__version__)
%store -r train_dir
%store -r validation_dir
%store -r eval_dir
%store -r data_dir | _____no_output_____ | MIT | code/phase0/1.2.Train_Keras_Local_Script_Mode.ipynb | gonsoomoon-ml/SageMaker-Tensorflow-Step-By-Step |
2. 노트북에서 세이지 메이커 스크립트 모드 스타일로 코드 변경- 이 페이지를 보시면 기본 코드를 세이지 메이커 스크립트 모드로 변경하는 내용이 있습니다. - [Train a Keras Sequential Model (TensorFlow 2.0)](https://github.com/daekeun-ml/tensorflow-in-sagemaker-workshop/blob/master/0_Running_TensorFlow_In_SageMaker_tf2.ipynb)- 아래의 ` !pygmentize src/cifar10_keras_sm_tf2.py` 의 주석을 제거하시고 보시면 실제 변경된 코드가 있습니다. | # !pygmentize src/cifar10_keras_sm_tf2.py | _____no_output_____ | MIT | code/phase0/1.2.Train_Keras_Local_Script_Mode.ipynb | gonsoomoon-ml/SageMaker-Tensorflow-Step-By-Step |
3. 세이지 메이커 스크립트 모드 스타일로 코드 실행 (실제로 세이지 메이커 사용 안함)테스트를 위해 위와 동일한 명령(command)으로 새 스크립트를 실행하고, 예상대로 실행되는지 확인합니다. SageMaker TensorFlow API 호출 시에 환경 변수들은 자동으로 넘겨기지만, 로컬 주피터 노트북에서 테스트 시에는 수동으로 환경 변수들을 지정해야 합니다. (아래 예제 코드를 참조해 주세요.)```python%env SM_MODEL_DIR=./logs``` | print("train_dir: ", train_dir)
print("validation_dir: ", validation_dir)
print("eval_dir: ", eval_dir)
%%time
!mkdir -p logs
# Number of GPUs on this machine
%env SM_NUM_GPUS=1
# Where to save the model
%env SM_MODEL_DIR=./logs
!python src/cifar10_keras_sm_tf2.py --model_dir ./logs \
--train {train_dir} \
--validation {validation_dir} \
--eval {eval_dir} \
--epochs 1
!rm -rf logs | env: SM_NUM_GPUS=1
env: SM_MODEL_DIR=./logs
args:
Namespace(batch_size=128, epochs=1, eval='data/cifar10/eval', learning_rate=0.001, model_dir='./logs', model_output_dir='./logs', momentum=0.9, optimizer='adam', train='data/cifar10/train', validation='data/cifar10/validation', weight_decay=0.0002)
312/312 [==============================] - 76s 237ms/step - loss: 2.2003 - accuracy: 0.2331 - val_loss: 1.8217 - val_accuracy: 0.3403
INFO:tensorflow:Assets written to: ./logs/1/assets
INFO:tensorflow:Assets written to: ./logs/1/assets
CPU times: user 2.05 s, sys: 316 ms, total: 2.37 s
Wall time: 1min 27s
| MIT | code/phase0/1.2.Train_Keras_Local_Script_Mode.ipynb | gonsoomoon-ml/SageMaker-Tensorflow-Step-By-Step |
4. 세이지 메이커 로컬 모드로 훈련본격적으로 학습을 시작하기 전에 로컬 모드를 사용하여 디버깅을 먼저 수행합니다. 로컬 모드는 학습 인스턴스를 생성하는 과정이 없이 로컬 인스턴스로 컨테이너를 가져온 후 곧바로 학습을 수행하기 때문에 코드를 보다 신속히 검증할 수 있습니다.Amazon SageMaker Python SDK의 로컬 모드는 TensorFlow 또는 MXNet estimator서 단일 인자값을 변경하여 CPU (단일 및 다중 인스턴스) 및 GPU (단일 인스턴스) SageMaker 학습 작업을 에뮬레이션(enumlate)할 수 있습니다. 로컬 모드 학습을 위해서는 docker-compose 또는 nvidia-docker-compose (GPU 인스턴스인 경우)의 설치가 필요합니다. 아래 코드 셀을 통해 본 노트북 환경에 docker-compose 또는 nvidia-docker-compose를 설치하고 구성합니다. 로컬 모드의 학습을 통해 여러분의 코드가 현재 사용 중인 하드웨어를 적절히 활용하고 있는지 확인하기 위한 GPU 점유와 같은 지표(metric)를 쉽게 모니터링할 수 있습니다. | !wget -q https://raw.githubusercontent.com/aws-samples/amazon-sagemaker-script-mode/master/local_mode_setup.sh
!wget -q https://raw.githubusercontent.com/aws-samples/amazon-sagemaker-script-mode/master/daemon.json
!/bin/bash ./local_mode_setup.sh | nvidia-docker2 already installed. We are good to go!
SageMaker instance route table setup is ok. We are good to go.
SageMaker instance routing for Docker is ok. We are good to go!
| MIT | code/phase0/1.2.Train_Keras_Local_Script_Mode.ipynb | gonsoomoon-ml/SageMaker-Tensorflow-Step-By-Step |
로컬 모드로 훈련 실행- 아래의 두 라인이 로컬모드로 훈련을 지시 합니다.```python instance_type=instance_type, local_gpu or local 지정 session = sagemaker.LocalSession(), 로컬 세션을 사용합니다.``` 로컬의 GPU, CPU 여부로 instance_type 결정 | import os
import subprocess
instance_type = "local_gpu" # GPU 사용을 가정 합니다. CPU 사용시에 'local' 로 정의 합니다.
print("Instance type = " + instance_type) | Instance type = local_gpu
| MIT | code/phase0/1.2.Train_Keras_Local_Script_Mode.ipynb | gonsoomoon-ml/SageMaker-Tensorflow-Step-By-Step |
학습 작업을 시작하기 위해 `estimator.fit() ` 호출 시, Amazon ECR에서 Amazon SageMaker TensorFlow 컨테이너를 로컬 노트북 인스턴스로 다운로드합니다.`sagemaker.tensorflow` 클래스를 사용하여 SageMaker Python SDK의 Tensorflow Estimator 인스턴스를 생성합니다.인자값으로 하이퍼파라메터와 다양한 설정들을 변경할 수 있습니다.자세한 내용은 [documentation](https://sagemaker.readthedocs.io/en/stable/using_tf.htmltraining-with-tensorflow-estimator)을 확인하시기 바랍니다. | from sagemaker.tensorflow import TensorFlow
estimator = TensorFlow(base_job_name='cifar10',
entry_point='cifar10_keras_sm_tf2.py',
source_dir='src',
role=role,
framework_version='2.4.1',
py_version='py37',
script_mode=True,
hyperparameters={'epochs' : 1},
train_instance_count=1,
train_instance_type= instance_type)
%%time
estimator.fit({'train': f'file://{train_dir}',
'validation': f'file://{validation_dir}',
'eval': f'file://{eval_dir}'}) | Creating roqiryt46i-algo-1-jt4ft ...
Creating roqiryt46i-algo-1-jt4ft ... done
Attaching to roqiryt46i-algo-1-jt4ft
[36mroqiryt46i-algo-1-jt4ft |[0m 2021-10-11 09:57:34.729714: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.
[36mroqiryt46i-algo-1-jt4ft |[0m 2021-10-11 09:57:34.729908: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:105] SageMaker Profiler is not enabled. The timeline writer thread will not be started, future recorded events will be dropped.
[36mroqiryt46i-algo-1-jt4ft |[0m 2021-10-11 09:57:34.734209: I tensorflow/stream_executor/platform/default/dso_loader.cc:49] Successfully opened dynamic library libcudart.so.11.0
[36mroqiryt46i-algo-1-jt4ft |[0m 2021-10-11 09:57:34.771766: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.
[36mroqiryt46i-algo-1-jt4ft |[0m 2021-10-11 09:57:36,568 sagemaker-training-toolkit INFO Imported framework sagemaker_tensorflow_container.training
[36mroqiryt46i-algo-1-jt4ft |[0m 2021-10-11 09:57:36,940 sagemaker-training-toolkit INFO Invoking user script
[36mroqiryt46i-algo-1-jt4ft |[0m
[36mroqiryt46i-algo-1-jt4ft |[0m Training Env:
[36mroqiryt46i-algo-1-jt4ft |[0m
[36mroqiryt46i-algo-1-jt4ft |[0m {
[36mroqiryt46i-algo-1-jt4ft |[0m "additional_framework_parameters": {},
[36mroqiryt46i-algo-1-jt4ft |[0m "channel_input_dirs": {
[36mroqiryt46i-algo-1-jt4ft |[0m "train": "/opt/ml/input/data/train",
[36mroqiryt46i-algo-1-jt4ft |[0m "validation": "/opt/ml/input/data/validation",
[36mroqiryt46i-algo-1-jt4ft |[0m "eval": "/opt/ml/input/data/eval"
[36mroqiryt46i-algo-1-jt4ft |[0m },
[36mroqiryt46i-algo-1-jt4ft |[0m "current_host": "algo-1-jt4ft",
[36mroqiryt46i-algo-1-jt4ft |[0m "framework_module": "sagemaker_tensorflow_container.training:main",
[36mroqiryt46i-algo-1-jt4ft |[0m "hosts": [
[36mroqiryt46i-algo-1-jt4ft |[0m "algo-1-jt4ft"
[36mroqiryt46i-algo-1-jt4ft |[0m ],
[36mroqiryt46i-algo-1-jt4ft |[0m "hyperparameters": {
[36mroqiryt46i-algo-1-jt4ft |[0m "epochs": 1,
[36mroqiryt46i-algo-1-jt4ft |[0m "model_dir": "s3://sagemaker-us-east-1-227612457811/cifar10-2021-10-11-09-53-58-320/model"
[36mroqiryt46i-algo-1-jt4ft |[0m },
[36mroqiryt46i-algo-1-jt4ft |[0m "input_config_dir": "/opt/ml/input/config",
[36mroqiryt46i-algo-1-jt4ft |[0m "input_data_config": {
[36mroqiryt46i-algo-1-jt4ft |[0m "train": {
[36mroqiryt46i-algo-1-jt4ft |[0m "TrainingInputMode": "File"
[36mroqiryt46i-algo-1-jt4ft |[0m },
[36mroqiryt46i-algo-1-jt4ft |[0m "validation": {
[36mroqiryt46i-algo-1-jt4ft |[0m "TrainingInputMode": "File"
[36mroqiryt46i-algo-1-jt4ft |[0m },
[36mroqiryt46i-algo-1-jt4ft |[0m "eval": {
[36mroqiryt46i-algo-1-jt4ft |[0m "TrainingInputMode": "File"
[36mroqiryt46i-algo-1-jt4ft |[0m }
[36mroqiryt46i-algo-1-jt4ft |[0m },
[36mroqiryt46i-algo-1-jt4ft |[0m "input_dir": "/opt/ml/input",
[36mroqiryt46i-algo-1-jt4ft |[0m "is_master": true,
[36mroqiryt46i-algo-1-jt4ft |[0m "job_name": "cifar10-2021-10-11-09-53-58-320",
[36mroqiryt46i-algo-1-jt4ft |[0m "log_level": 20,
[36mroqiryt46i-algo-1-jt4ft |[0m "master_hostname": "algo-1-jt4ft",
[36mroqiryt46i-algo-1-jt4ft |[0m "model_dir": "/opt/ml/model",
[36mroqiryt46i-algo-1-jt4ft |[0m "module_dir": "s3://sagemaker-us-east-1-227612457811/cifar10-2021-10-11-09-53-58-320/source/sourcedir.tar.gz",
[36mroqiryt46i-algo-1-jt4ft |[0m "module_name": "cifar10_keras_sm_tf2",
[36mroqiryt46i-algo-1-jt4ft |[0m "network_interface_name": "eth0",
[36mroqiryt46i-algo-1-jt4ft |[0m "num_cpus": 8,
[36mroqiryt46i-algo-1-jt4ft |[0m "num_gpus": 1,
[36mroqiryt46i-algo-1-jt4ft |[0m "output_data_dir": "/opt/ml/output/data",
[36mroqiryt46i-algo-1-jt4ft |[0m "output_dir": "/opt/ml/output",
[36mroqiryt46i-algo-1-jt4ft |[0m "output_intermediate_dir": "/opt/ml/output/intermediate",
[36mroqiryt46i-algo-1-jt4ft |[0m "resource_config": {
[36mroqiryt46i-algo-1-jt4ft |[0m "current_host": "algo-1-jt4ft",
[36mroqiryt46i-algo-1-jt4ft |[0m "hosts": [
[36mroqiryt46i-algo-1-jt4ft |[0m "algo-1-jt4ft"
[36mroqiryt46i-algo-1-jt4ft |[0m ]
[36mroqiryt46i-algo-1-jt4ft |[0m },
[36mroqiryt46i-algo-1-jt4ft |[0m "user_entry_point": "cifar10_keras_sm_tf2.py"
[36mroqiryt46i-algo-1-jt4ft |[0m }
[36mroqiryt46i-algo-1-jt4ft |[0m
[36mroqiryt46i-algo-1-jt4ft |[0m Environment variables:
[36mroqiryt46i-algo-1-jt4ft |[0m
[36mroqiryt46i-algo-1-jt4ft |[0m SM_HOSTS=["algo-1-jt4ft"]
[36mroqiryt46i-algo-1-jt4ft |[0m SM_NETWORK_INTERFACE_NAME=eth0
[36mroqiryt46i-algo-1-jt4ft |[0m SM_HPS={"epochs":1,"model_dir":"s3://sagemaker-us-east-1-227612457811/cifar10-2021-10-11-09-53-58-320/model"}
[36mroqiryt46i-algo-1-jt4ft |[0m SM_USER_ENTRY_POINT=cifar10_keras_sm_tf2.py
[36mroqiryt46i-algo-1-jt4ft |[0m SM_FRAMEWORK_PARAMS={}
[36mroqiryt46i-algo-1-jt4ft |[0m SM_RESOURCE_CONFIG={"current_host":"algo-1-jt4ft","hosts":["algo-1-jt4ft"]}
[36mroqiryt46i-algo-1-jt4ft |[0m SM_INPUT_DATA_CONFIG={"eval":{"TrainingInputMode":"File"},"train":{"TrainingInputMode":"File"},"validation":{"TrainingInputMode":"File"}}
[36mroqiryt46i-algo-1-jt4ft |[0m SM_OUTPUT_DATA_DIR=/opt/ml/output/data
[36mroqiryt46i-algo-1-jt4ft |[0m SM_CHANNELS=["eval","train","validation"]
[36mroqiryt46i-algo-1-jt4ft |[0m SM_CURRENT_HOST=algo-1-jt4ft
[36mroqiryt46i-algo-1-jt4ft |[0m SM_MODULE_NAME=cifar10_keras_sm_tf2
[36mroqiryt46i-algo-1-jt4ft |[0m SM_LOG_LEVEL=20
[36mroqiryt46i-algo-1-jt4ft |[0m SM_FRAMEWORK_MODULE=sagemaker_tensorflow_container.training:main
[36mroqiryt46i-algo-1-jt4ft |[0m SM_INPUT_DIR=/opt/ml/input
[36mroqiryt46i-algo-1-jt4ft |[0m SM_INPUT_CONFIG_DIR=/opt/ml/input/config
[36mroqiryt46i-algo-1-jt4ft |[0m SM_OUTPUT_DIR=/opt/ml/output
[36mroqiryt46i-algo-1-jt4ft |[0m SM_NUM_CPUS=8
[36mroqiryt46i-algo-1-jt4ft |[0m SM_NUM_GPUS=1
[36mroqiryt46i-algo-1-jt4ft |[0m SM_MODEL_DIR=/opt/ml/model
[36mroqiryt46i-algo-1-jt4ft |[0m SM_MODULE_DIR=s3://sagemaker-us-east-1-227612457811/cifar10-2021-10-11-09-53-58-320/source/sourcedir.tar.gz
[36mroqiryt46i-algo-1-jt4ft |[0m SM_TRAINING_ENV={"additional_framework_parameters":{},"channel_input_dirs":{"eval":"/opt/ml/input/data/eval","train":"/opt/ml/input/data/train","validation":"/opt/ml/input/data/validation"},"current_host":"algo-1-jt4ft","framework_module":"sagemaker_tensorflow_container.training:main","hosts":["algo-1-jt4ft"],"hyperparameters":{"epochs":1,"model_dir":"s3://sagemaker-us-east-1-227612457811/cifar10-2021-10-11-09-53-58-320/model"},"input_config_dir":"/opt/ml/input/config","input_data_config":{"eval":{"TrainingInputMode":"File"},"train":{"TrainingInputMode":"File"},"validation":{"TrainingInputMode":"File"}},"input_dir":"/opt/ml/input","is_master":true,"job_name":"cifar10-2021-10-11-09-53-58-320","log_level":20,"master_hostname":"algo-1-jt4ft","model_dir":"/opt/ml/model","module_dir":"s3://sagemaker-us-east-1-227612457811/cifar10-2021-10-11-09-53-58-320/source/sourcedir.tar.gz","module_name":"cifar10_keras_sm_tf2","network_interface_name":"eth0","num_cpus":8,"num_gpus":1,"output_data_dir":"/opt/ml/output/data","output_dir":"/opt/ml/output","output_intermediate_dir":"/opt/ml/output/intermediate","resource_config":{"current_host":"algo-1-jt4ft","hosts":["algo-1-jt4ft"]},"user_entry_point":"cifar10_keras_sm_tf2.py"}
[36mroqiryt46i-algo-1-jt4ft |[0m SM_USER_ARGS=["--epochs","1","--model_dir","s3://sagemaker-us-east-1-227612457811/cifar10-2021-10-11-09-53-58-320/model"]
[36mroqiryt46i-algo-1-jt4ft |[0m SM_OUTPUT_INTERMEDIATE_DIR=/opt/ml/output/intermediate
[36mroqiryt46i-algo-1-jt4ft |[0m SM_CHANNEL_TRAIN=/opt/ml/input/data/train
[36mroqiryt46i-algo-1-jt4ft |[0m SM_CHANNEL_VALIDATION=/opt/ml/input/data/validation
[36mroqiryt46i-algo-1-jt4ft |[0m SM_CHANNEL_EVAL=/opt/ml/input/data/eval
[36mroqiryt46i-algo-1-jt4ft |[0m SM_HP_EPOCHS=1
[36mroqiryt46i-algo-1-jt4ft |[0m SM_HP_MODEL_DIR=s3://sagemaker-us-east-1-227612457811/cifar10-2021-10-11-09-53-58-320/model
[36mroqiryt46i-algo-1-jt4ft |[0m PYTHONPATH=/opt/ml/code:/usr/local/bin:/usr/local/lib/python37.zip:/usr/local/lib/python3.7:/usr/local/lib/python3.7/lib-dynload:/usr/local/lib/python3.7/site-packages
[36mroqiryt46i-algo-1-jt4ft |[0m
[36mroqiryt46i-algo-1-jt4ft |[0m Invoking script with the following command:
[36mroqiryt46i-algo-1-jt4ft |[0m
[36mroqiryt46i-algo-1-jt4ft |[0m /usr/local/bin/python3.7 cifar10_keras_sm_tf2.py --epochs 1 --model_dir s3://sagemaker-us-east-1-227612457811/cifar10-2021-10-11-09-53-58-320/model
[36mroqiryt46i-algo-1-jt4ft |[0m
[36mroqiryt46i-algo-1-jt4ft |[0m
[36mroqiryt46i-algo-1-jt4ft |[0m args:
[36mroqiryt46i-algo-1-jt4ft |[0m Namespace(batch_size=128, epochs=1, eval='/opt/ml/input/data/eval', learning_rate=0.001, model_dir='s3://sagemaker-us-east-1-227612457811/cifar10-2021-10-11-09-53-58-320/model', model_output_dir='/opt/ml/model', momentum=0.9, optimizer='adam', train='/opt/ml/input/data/train', validation='/opt/ml/input/data/validation', weight_decay=0.0002)
[36mroqiryt46i-algo-1-jt4ft |[0m [2021-10-11 09:57:41.223 a4d4778958f9:37 INFO utils.py:27] RULE_JOB_STOP_SIGNAL_FILENAME: None
[36mroqiryt46i-algo-1-jt4ft |[0m [2021-10-11 09:57:41.329 a4d4778958f9:37 INFO profiler_config_parser.py:102] Unable to find config at /opt/ml/input/config/profilerconfig.json. Profiler is disabled.
312/312 [==============================] - 9s 14ms/step - loss: 2.1226 - accuracy: 0.2532 - val_loss: 1.8308 - val_accuracy: 0.3635
[36mroqiryt46i-algo-1-jt4ft |[0m 2021-10-11 09:57:37.091032: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.
[36mroqiryt46i-algo-1-jt4ft |[0m 2021-10-11 09:57:37.091215: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:105] SageMaker Profiler is not enabled. The timeline writer thread will not be started, future recorded events will be dropped.
[36mroqiryt46i-algo-1-jt4ft |[0m 2021-10-11 09:57:37.132764: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.
[36mroqiryt46i-algo-1-jt4ft |[0m 2021-10-11 09:57:52.594222: W tensorflow/python/util/util.cc:348] Sets are not currently considered sequences, but this may change in the future, so consider avoiding using them.
[36mroqiryt46i-algo-1-jt4ft |[0m INFO:tensorflow:Assets written to: /opt/ml/model/1/assets
[36mroqiryt46i-algo-1-jt4ft |[0m INFO:tensorflow:Assets written to: /opt/ml/model/1/assets
[36mroqiryt46i-algo-1-jt4ft |[0m
[36mroqiryt46i-algo-1-jt4ft |[0m 2021-10-11 09:57:56,497 sagemaker-training-toolkit INFO Reporting training SUCCESS
[36mroqiryt46i-algo-1-jt4ft exited with code 0
[0mAborting on container exit...
===== Job Complete =====
CPU times: user 1.07 s, sys: 126 ms, total: 1.19 s
Wall time: 4min
| MIT | code/phase0/1.2.Train_Keras_Local_Script_Mode.ipynb | gonsoomoon-ml/SageMaker-Tensorflow-Step-By-Step |
ECR 로 부터 로컬에 다운로드된 도커 이미지 확인 | ! docker image ls | REPOSITORY TAG IMAGE ID CREATED SIZE
763104351884.dkr.ecr.us-east-1.amazonaws.com/tensorflow-training 2.4.1-gpu-py37 8467bc1c5070 5 months ago 8.91GB
| MIT | code/phase0/1.2.Train_Keras_Local_Script_Mode.ipynb | gonsoomoon-ml/SageMaker-Tensorflow-Step-By-Step |
5. 세이지 메이커의 호스트 모드로 훈련 데이터 세트를 S3에 업로드- 로컬에 저장되어 있는 데이터를 S3 로 업로드하여 사용합니다. | dataset_location = sagemaker_session.upload_data(path=data_dir, key_prefix='data/DEMO-cifar10')
display(dataset_location)
from sagemaker.tensorflow import TensorFlow
estimator = TensorFlow(base_job_name='cifar10',
entry_point='cifar10_keras_sm_tf2.py',
source_dir='src',
role=role,
framework_version='2.4.1',
py_version='py37',
script_mode=True,
hyperparameters={'epochs': 5},
train_instance_count=1,
train_instance_type='ml.p2.8xlarge') | train_instance_type has been renamed in sagemaker>=2.
See: https://sagemaker.readthedocs.io/en/stable/v2.html for details.
train_instance_count has been renamed in sagemaker>=2.
See: https://sagemaker.readthedocs.io/en/stable/v2.html for details.
train_instance_type has been renamed in sagemaker>=2.
See: https://sagemaker.readthedocs.io/en/stable/v2.html for details.
| MIT | code/phase0/1.2.Train_Keras_Local_Script_Mode.ipynb | gonsoomoon-ml/SageMaker-Tensorflow-Step-By-Step |
SageMaker Host Mode 로 훈련- `cifar10_estimator.fit(inputs, wait=False)` - 입력 데이터를 inputs로서 S3 의 경로를 제공합니다. - wait=False 로 지정해서 async 모드로 훈련을 실행합니다. - 실행 경과는 아래의 cifar10_estimator.logs() 에서 확인 합니다. | %%time
estimator.fit({'train':'{}/train'.format(dataset_location),
'validation':'{}/validation'.format(dataset_location),
'eval':'{}/eval'.format(dataset_location)}, wait=False)
estimator.logs() | 2021-10-11 10:10:06 Starting - Starting the training job...
2021-10-11 10:10:29 Starting - Launching requested ML instancesProfilerReport-1633947005: InProgress
............
2021-10-11 10:12:29 Starting - Preparing the instances for training.........
2021-10-11 10:14:02 Downloading - Downloading input data
2021-10-11 10:14:02 Training - Downloading the training image..................
2021-10-11 10:17:00 Training - Training image download completed. Training in progress.[34m2021-10-11 10:17:01.282337: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m2021-10-11 10:17:01.288520: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:105] SageMaker Profiler is not enabled. The timeline writer thread will not be started, future recorded events will be dropped.[0m
[34m2021-10-11 10:17:01.384938: I tensorflow/stream_executor/platform/default/dso_loader.cc:49] Successfully opened dynamic library libcudart.so.11.0[0m
[34m2021-10-11 10:17:01.489458: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m2021-10-11 10:17:06,008 sagemaker-training-toolkit INFO Imported framework sagemaker_tensorflow_container.training[0m
[34m2021-10-11 10:17:06,769 sagemaker-training-toolkit INFO Invoking user script
[0m
[34mTraining Env:
[0m
[34m{
"additional_framework_parameters": {},
"channel_input_dirs": {
"eval": "/opt/ml/input/data/eval",
"validation": "/opt/ml/input/data/validation",
"train": "/opt/ml/input/data/train"
},
"current_host": "algo-1",
"framework_module": "sagemaker_tensorflow_container.training:main",
"hosts": [
"algo-1"
],
"hyperparameters": {
"model_dir": "s3://sagemaker-us-east-1-227612457811/cifar10-2021-10-11-09-58-44-104/model",
"epochs": 5
},
"input_config_dir": "/opt/ml/input/config",
"input_data_config": {
"eval": {
"TrainingInputMode": "File",
"S3DistributionType": "FullyReplicated",
"RecordWrapperType": "None"
},
"validation": {
"TrainingInputMode": "File",
"S3DistributionType": "FullyReplicated",
"RecordWrapperType": "None"
},
"train": {
"TrainingInputMode": "File",
"S3DistributionType": "FullyReplicated",
"RecordWrapperType": "None"
}
},
"input_dir": "/opt/ml/input",
"is_master": true,
"job_name": "cifar10-2021-10-11-10-10-05-339",
"log_level": 20,
"master_hostname": "algo-1",
"model_dir": "/opt/ml/model",
"module_dir": "s3://sagemaker-us-east-1-227612457811/cifar10-2021-10-11-10-10-05-339/source/sourcedir.tar.gz",
"module_name": "cifar10_keras_sm_tf2",
"network_interface_name": "eth0",
"num_cpus": 32,
"num_gpus": 8,
"output_data_dir": "/opt/ml/output/data",
"output_dir": "/opt/ml/output",
"output_intermediate_dir": "/opt/ml/output/intermediate",
"resource_config": {
"current_host": "algo-1",
"hosts": [
"algo-1"
],
"network_interface_name": "eth0"
},
"user_entry_point": "cifar10_keras_sm_tf2.py"[0m
[34m}
[0m
[34mEnvironment variables:
[0m
[34mSM_HOSTS=["algo-1"][0m
[34mSM_NETWORK_INTERFACE_NAME=eth0[0m
[34mSM_HPS={"epochs":5,"model_dir":"s3://sagemaker-us-east-1-227612457811/cifar10-2021-10-11-09-58-44-104/model"}[0m
[34mSM_USER_ENTRY_POINT=cifar10_keras_sm_tf2.py[0m
[34mSM_FRAMEWORK_PARAMS={}[0m
[34mSM_RESOURCE_CONFIG={"current_host":"algo-1","hosts":["algo-1"],"network_interface_name":"eth0"}[0m
[34mSM_INPUT_DATA_CONFIG={"eval":{"RecordWrapperType":"None","S3DistributionType":"FullyReplicated","TrainingInputMode":"File"},"train":{"RecordWrapperType":"None","S3DistributionType":"FullyReplicated","TrainingInputMode":"File"},"validation":{"RecordWrapperType":"None","S3DistributionType":"FullyReplicated","TrainingInputMode":"File"}}[0m
[34mSM_OUTPUT_DATA_DIR=/opt/ml/output/data[0m
[34mSM_CHANNELS=["eval","train","validation"][0m
[34mSM_CURRENT_HOST=algo-1[0m
[34mSM_MODULE_NAME=cifar10_keras_sm_tf2[0m
[34mSM_LOG_LEVEL=20[0m
[34mSM_FRAMEWORK_MODULE=sagemaker_tensorflow_container.training:main[0m
[34mSM_INPUT_DIR=/opt/ml/input[0m
[34mSM_INPUT_CONFIG_DIR=/opt/ml/input/config[0m
[34mSM_OUTPUT_DIR=/opt/ml/output[0m
[34mSM_NUM_CPUS=32[0m
[34mSM_NUM_GPUS=8[0m
[34mSM_MODEL_DIR=/opt/ml/model[0m
[34mSM_MODULE_DIR=s3://sagemaker-us-east-1-227612457811/cifar10-2021-10-11-10-10-05-339/source/sourcedir.tar.gz[0m
[34mSM_TRAINING_ENV={"additional_framework_parameters":{},"channel_input_dirs":{"eval":"/opt/ml/input/data/eval","train":"/opt/ml/input/data/train","validation":"/opt/ml/input/data/validation"},"current_host":"algo-1","framework_module":"sagemaker_tensorflow_container.training:main","hosts":["algo-1"],"hyperparameters":{"epochs":5,"model_dir":"s3://sagemaker-us-east-1-227612457811/cifar10-2021-10-11-09-58-44-104/model"},"input_config_dir":"/opt/ml/input/config","input_data_config":{"eval":{"RecordWrapperType":"None","S3DistributionType":"FullyReplicated","TrainingInputMode":"File"},"train":{"RecordWrapperType":"None","S3DistributionType":"FullyReplicated","TrainingInputMode":"File"},"validation":{"RecordWrapperType":"None","S3DistributionType":"FullyReplicated","TrainingInputMode":"File"}},"input_dir":"/opt/ml/input","is_master":true,"job_name":"cifar10-2021-10-11-10-10-05-339","log_level":20,"master_hostname":"algo-1","model_dir":"/opt/ml/model","module_dir":"s3://sagemaker-us-east-1-227612457811/cifar10-2021-10-11-10-10-05-339/source/sourcedir.tar.gz","module_name":"cifar10_keras_sm_tf2","network_interface_name":"eth0","num_cpus":32,"num_gpus":8,"output_data_dir":"/opt/ml/output/data","output_dir":"/opt/ml/output","output_intermediate_dir":"/opt/ml/output/intermediate","resource_config":{"current_host":"algo-1","hosts":["algo-1"],"network_interface_name":"eth0"},"user_entry_point":"cifar10_keras_sm_tf2.py"}[0m
[34mSM_USER_ARGS=["--epochs","5","--model_dir","s3://sagemaker-us-east-1-227612457811/cifar10-2021-10-11-09-58-44-104/model"][0m
[34mSM_OUTPUT_INTERMEDIATE_DIR=/opt/ml/output/intermediate[0m
[34mSM_CHANNEL_EVAL=/opt/ml/input/data/eval[0m
[34mSM_CHANNEL_VALIDATION=/opt/ml/input/data/validation[0m
[34mSM_CHANNEL_TRAIN=/opt/ml/input/data/train[0m
[34mSM_HP_MODEL_DIR=s3://sagemaker-us-east-1-227612457811/cifar10-2021-10-11-09-58-44-104/model[0m
[34mSM_HP_EPOCHS=5[0m
[34mPYTHONPATH=/opt/ml/code:/usr/local/bin:/usr/local/lib/python37.zip:/usr/local/lib/python3.7:/usr/local/lib/python3.7/lib-dynload:/usr/local/lib/python3.7/site-packages
[0m
[34mInvoking script with the following command:
[0m
[34m/usr/local/bin/python3.7 cifar10_keras_sm_tf2.py --epochs 5 --model_dir s3://sagemaker-us-east-1-227612457811/cifar10-2021-10-11-09-58-44-104/model
[0m
[34margs:
Namespace(batch_size=128, epochs=5, eval='/opt/ml/input/data/eval', learning_rate=0.001, model_dir='s3://sagemaker-us-east-1-227612457811/cifar10-2021-10-11-09-58-44-104/model', model_output_dir='/opt/ml/model', momentum=0.9, optimizer='adam', train='/opt/ml/input/data/train', validation='/opt/ml/input/data/validation', weight_decay=0.0002)[0m
[34m[2021-10-11 10:17:17.171 ip-10-0-206-213.ec2.internal:82 INFO utils.py:27] RULE_JOB_STOP_SIGNAL_FILENAME: None[0m
[34m[2021-10-11 10:17:17.324 ip-10-0-206-213.ec2.internal:82 INFO profiler_config_parser.py:102] User has disabled profiler.[0m
[34m[2021-10-11 10:17:17.701 ip-10-0-206-213.ec2.internal:82 INFO json_config.py:91] Creating hook from json_config at /opt/ml/input/config/debughookconfig.json.[0m
[34m[2021-10-11 10:17:17.701 ip-10-0-206-213.ec2.internal:82 INFO hook.py:199] tensorboard_dir has not been set for the hook. SMDebug will not be exporting tensorboard summaries.[0m
[34m[2021-10-11 10:17:17.702 ip-10-0-206-213.ec2.internal:82 INFO hook.py:253] Saving to /opt/ml/output/tensors[0m
[34m[2021-10-11 10:17:17.702 ip-10-0-206-213.ec2.internal:82 INFO state_store.py:77] The checkpoint config file /opt/ml/input/config/checkpointconfig.json does not exist.[0m
[34mEpoch 1/5[0m
[34m[2021-10-11 10:17:17.751 ip-10-0-206-213.ec2.internal:82 INFO hook.py:413] Monitoring the collections: metrics, sm_metrics, losses[0m
[34m#015 1/312 [..............................] - ETA: 59:33 - loss: 4.1698 - accuracy: 0.0547#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 2/312 [..............................] - ETA: 1:52 - loss: 3.9593 - accuracy: 0.0703 #010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 3/312 [..............................] - ETA: 1:16 - loss: 3.8742 - accuracy: 0.0764#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 4/312 [..............................] - ETA: 1:03 - loss: 3.8124 - accuracy: 0.0817#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 5/312 [..............................] - ETA: 53s - loss: 3.7526 - accuracy: 0.0869 #010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 6/312 [..............................] - ETA: 47s - loss: 3.6985 - accuracy: 0.0907#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 7/312 [..............................] - ETA: 42s - loss: 3.6438 - accuracy: 0.0938#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 8/312 [..............................] - ETA: 39s - loss: 3.5947 - accuracy: 0.0966#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 10/312 [..............................] - ETA: 33s - loss: 3.5067 - accuracy: 0.1011#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 12/312 [>.............................] - ETA: 29s - loss: 3.4288 - accuracy: 0.1060#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 14/312 [>.............................] - ETA: 26s - loss: 3.3607 - accuracy: 0.1106#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 16/312 [>.............................] - ETA: 23s - loss: 3.2987 - accuracy: 0.1144#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 18/312 [>.............................] - ETA: 21s - loss: 3.2426 - accuracy: 0.1180#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 20/312 [>.............................] - ETA: 20s - loss: 3.1924 - accuracy: 0.1211#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 22/312 [=>............................] - ETA: 19s - loss: 3.1469 - accuracy: 0.1240#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 24/312 [=>............................] - ETA: 18s - loss: 3.1051 - accuracy: 0.1268#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 26/312 [=>............................] - ETA: 17s - loss: 3.0664 - accuracy: 0.1296#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 28/312 [=>............................] - ETA: 16s - loss: 3.0311 - accuracy: 0.1323#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 30/312 [=>............................] - ETA: 15s - loss: 2.9987 - accuracy: 0.1349#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 32/312 [==>...........................] - ETA: 15s - loss: 2.9686 - accuracy: 0.1373#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 34/312 [==>...........................] - ETA: 14s - loss: 2.9405 - accuracy: 0.1396#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 36/312 [==>...........................] - ETA: 14s - loss: 2.9142 - accuracy: 0.1418#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 38/312 [==>...........................] - ETA: 13s - loss: 2.8898 - accuracy: 0.1439#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 40/312 [==>...........................] - ETA: 13s - loss: 2.8670 - accuracy: 0.1459#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 42/312 [===>..........................] - ETA: 12s - loss: 2.8454 - accuracy: 0.1479#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 44/312 [===>..........................] - ETA: 12s - loss: 2.8251 - accuracy: 0.1497#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 46/312 [===>..........................] - ETA: 12s - loss: 2.8058 - accuracy: 0.1515#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 48/312 [===>..........................] - ETA: 12s - loss: 2.7876 - accuracy: 0.1531#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 50/312 [===>..........................] - ETA: 11s - loss: 2.7702 - accuracy: 0.1548#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 52/312 [====>.........................] - ETA: 11s - loss: 2.7537 - accuracy: 0.1564#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 54/312 [====>.........................] - ETA: 11s - loss: 2.7379 - accuracy: 0.1580#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 56/312 [====>.........................] - ETA: 11s - loss: 2.7228 - accuracy: 0.1596#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 58/312 [====>.........................] - ETA: 10s - loss: 2.7084 - accuracy: 0.1612#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 60/312 [====>.........................] - ETA: 10s - loss: 2.6945 - accuracy: 0.1627#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 62/312 [====>.........................] - ETA: 10s - loss: 2.6812 - accuracy: 0.1640#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 64/312 [=====>........................] - ETA: 10s - loss: 2.6683 - accuracy: 0.1654#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 66/312 [=====>........................] - ETA: 10s - loss: 2.6559 - accuracy: 0.1667#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 68/312 [=====>........................] - ETA: 9s - loss: 2.6440 - accuracy: 0.1679 #010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 70/312 [=====>........................] - ETA: 9s - loss: 2.6326 - accuracy: 0.1692#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 72/312 [=====>........................] - ETA: 9s - loss: 2.6216 - accuracy: 0.1704#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 74/312 [======>.......................] - ETA: 9s - loss: 2.6110 - accuracy: 0.1716#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 76/312 [======>.......................] - ETA: 9s - loss: 2.6007 - accuracy: 0.1727#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 78/312 [======>.......................] - ETA: 9s - loss: 2.5908 - accuracy: 0.1738#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 80/312 [======>.......................] - ETA: 9s - loss: 2.5811 - accuracy: 0.1749#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 82/312 [======>.......................] - ETA: 8s - loss: 2.5718 - accuracy: 0.1760#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 84/312 [=======>......................] - ETA: 8s - loss: 2.5627 - accuracy: 0.1771#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 86/312 [=======>......................] - ETA: 8s - loss: 2.5540 - accuracy: 0.1782#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 88/312 [=======>......................] - ETA: 8s - loss: 2.5455 - accuracy: 0.1792#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 90/312 [=======>......................] - ETA: 8s - loss: 2.5373 - accuracy: 0.1802#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 92/312 [=======>......................] - ETA: 8s - loss: 2.5293 - accuracy: 0.1812#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 94/312 [========>.....................] - ETA: 8s - loss: 2.5216 - accuracy: 0.1822#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 96/312 [========>.....................] - ETA: 8s - loss: 2.5140 - accuracy: 0.1831#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 98/312 [========>.....................] - ETA: 7s - loss: 2.5066 - accuracy: 0.1840#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015100/312 [========>.....................] - ETA: 7s - loss: 2.4994 - accuracy: 0.1849#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015102/312 [========>.....................] - ETA: 7s - loss: 2.4924 - accuracy: 0.1858#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015104/312 [=========>....................] - ETA: 7s - loss: 2.4855 - accuracy: 0.1868#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015106/312 [=========>....................] - ETA: 7s - loss: 2.4788 - accuracy: 0.1877#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015108/312 [=========>....................] - ETA: 7s - loss: 2.4722 - accuracy: 0.1885#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015110/312 [=========>....................] - ETA: 7s - loss: 2.4658 - accuracy: 0.1894#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015112/312 [=========>....................] - ETA: 7s - loss: 2.4596 - accuracy: 0.1903#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015114/312 [=========>....................] - ETA: 7s - loss: 2.4535 - accuracy: 0.1911#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015116/312 [==========>...................] - ETA: 7s - loss: 2.4475 - accuracy: 0.1920#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015118/312 [==========>...................] - ETA: 6s - loss: 2.4417 - accuracy: 0.1928#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015120/312 [==========>...................] - ETA: 6s - loss: 2.4359 - accuracy: 0.1936#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015122/312 [==========>...................] - ETA: 6s - loss: 2.4303 - accuracy: 0.1944#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015124/312 [==========>...................] - ETA: 6s - loss: 2.4248 - accuracy: 0.1952#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015126/312 [===========>..................] - ETA: 6s - loss: 2.4194 - accuracy: 0.1960#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015128/312 [===========>..................] - ETA: 6s - loss: 2.4141 - accuracy: 0.1968#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015130/312 [===========>..................] - ETA: 6s - loss: 2.4089 - accuracy: 0.1976#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015132/312 [===========>..................] - ETA: 6s - loss: 2.4037 - accuracy: 0.1984#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015134/312 [===========>..................] - ETA: 6s - loss: 2.3988 - accuracy: 0.1992#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015136/312 [============>.................] - ETA: 6s - loss: 2.3938 - accuracy: 0.1999#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015138/312 [============>.................] - ETA: 6s - loss: 2.3890 - accuracy: 0.2007#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015140/312 [============>.................] - ETA: 5s - loss: 2.3843 - accuracy: 0.2014#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015142/312 [============>.................] - ETA: 5s - loss: 2.3796 - accuracy: 0.2022#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015144/312 [============>.................] - ETA: 5s - loss: 2.3750 - accuracy: 0.2029#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015146/312 [=============>................] - ETA: 5s - loss: 2.3705 - accuracy: 0.2036#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015148/312 [=============>................] - ETA: 5s - loss: 2.3661 - accuracy: 0.2043#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015150/312 [=============>................] - ETA: 5s - loss: 2.3617 - accuracy: 0.2050#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015152/312 [=============>................] - ETA: 5s - loss: 2.3574 - accuracy: 0.2057#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015154/312 [=============>................] - ETA: 5s - loss: 2.3532 - accuracy: 0.2064#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015156/312 [==============>...............] - ETA: 5s - loss: 2.3490 - accuracy: 0.2071#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015158/312 [==============>...............] - ETA: 5s - loss: 2.3449 - accuracy: 0.2078#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015160/312 [==============>...............] - ETA: 5s - loss: 2.3408 - accuracy: 0.2085#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015162/312 [==============>...............] - ETA: 5s - loss: 2.3368 - accuracy: 0.2091#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015164/312 [==============>...............] - ETA: 5s - loss: 2.3328 - accuracy: 0.2098#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015166/312 [==============>...............] - ETA: 4s - loss: 2.3289 - accuracy: 0.2105#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015168/312 [===============>..............] - ETA: 4s - loss: 2.3251 - accuracy: 0.2111#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015170/312 [===============>..............] - ETA: 4s - loss: 2.3213 - accuracy: 0.2118#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015172/312 [===============>..............] - ETA: 4s - loss: 2.3176 - accuracy: 0.2124#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015174/312 [===============>..............] - ETA: 4s - loss: 2.3139 - accuracy: 0.2131#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015176/312 [===============>..............] - ETA: 4s - loss: 2.3102 - accuracy: 0.2137#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015178/312 [================>.............] - ETA: 4s - loss: 2.3066 - accuracy: 0.2144#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015180/312 [================>.............] - ETA: 4s - loss: 2.3031 - accuracy: 0.2150#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015182/312 [================>.............] - ETA: 4s - loss: 2.2996 - accuracy: 0.2156#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015184/312 [================>.............] - ETA: 4s - loss: 2.2961 - accuracy: 0.2163#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015186/312 [================>.............] - ETA: 4s - loss: 2.2927 - accu[0m
[34mracy: 0.2169#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015188/312 [=================>............] - ETA: 4s - loss: 2.2893 - accuracy: 0.2175#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015190/312 [=================>............] - ETA: 4s - loss: 2.2860 - accuracy: 0.2181#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015192/312 [=================>............] - ETA: 3s - loss: 2.2827 - accuracy: 0.2188#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015194/312 [=================>............] - ETA: 3s - loss: 2.2794 - accuracy: 0.2194#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015196/312 [=================>............] - ETA: 3s - loss: 2.2762 - accuracy: 0.2200#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015198/312 [==================>...........] - ETA: 3s - loss: 2.2730 - accuracy: 0.2206#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015200/312 [==================>...........] - ETA: 3s - loss: 2.2699 - accuracy: 0.2212#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015202/312 [==================>...........] - ETA: 3s - loss: 2.2668 - accuracy: 0.2218#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015204/312 [==================>...........] - ETA: 3s - loss: 2.2637 - accuracy: 0.2224#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015206/312 [==================>...........] - ETA: 3s - loss: 2.2607 - accuracy: 0.2230#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015208/312 [===================>..........] - ETA: 3s - loss: 2.2577 - accuracy: 0.2236#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015210/312 [===================>..........] - ETA: 3s - loss: 2.2547 - accuracy: 0.2242#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015212/312 [===================>..........] - ETA: 3s - loss: 2.2518 - accuracy: 0.2247#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015214/312 [===================>..........] - ETA: 3s - loss: 2.2489 - accuracy: 0.2253#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015216/312 [===================>..........] - ETA: 3s - loss: 2.2460 - accuracy: 0.2259#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015218/312 [===================>..........] - ETA: 3s - loss: 2.2432 - accuracy: 0.2264#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015220/312 [====================>.........] - ETA: 3s - loss: 2.2404 - accuracy: 0.2270#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015222/312 [====================>.........] - ETA: 2s - loss: 2.2376 - accuracy: 0.2276#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015224/312 [====================>.........] - ETA: 2s - loss: 2.2349 - accuracy: 0.2281#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015226/312 [====================>.........] - ETA: 2s - loss: 2.2322 - accuracy: 0.2287#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015228/312 [====================>.........] - ETA: 2s - loss: 2.2295 - accuracy: 0.2292#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015230/312 [=====================>........] - ETA: 2s - loss: 2.2268 - accuracy: 0.2298#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015232/312 [=====================>........] - ETA: 2s - loss: 2.2242 - accuracy: 0.2304#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015234/312 [=====================>........] - ETA: 2s - loss: 2.2216 - accuracy: 0.2309#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015236/312 [=====================>........] - ETA: 2s - loss: 2.2190 - accuracy: 0.2315#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015238/312 [=====================>........] - ETA: 2s - loss: 2.2164 - accuracy: 0.2320#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015240/312 [======================>.......] - ETA: 2s - loss: 2.2139 - accuracy: 0.2326#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015242/312 [======================>.......] - ETA: 2s - loss: 2.2113 - accuracy: 0.2331#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015244/312 [======================>.......] - ETA: 2s - loss: 2.2088 - accuracy: 0.2337#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015246/312 [======================>.......] - ETA: 2s - loss: 2.2064 - accuracy: 0.2342#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015248/312 [======================>.......] - ETA: 2s - loss: 2.2039 - accuracy: 0.2347#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015250/312 [=======================>......] - ETA: 1s - loss: 2.2014 - accuracy: 0.2353#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015252/312 [=======================>......] - ETA: 1s - loss: 2.1990 - accuracy: 0.2358#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015254/312 [=======================>......] - ETA: 1s - loss: 2.1966 - accuracy: 0.2364#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015256/312 [=======================>......] - ETA: 1s - loss: 2.1942 - accuracy: 0.2369#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015258/312 [=======================>......] - ETA: 1s - loss: 2.1918 - accuracy: 0.2374#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015260/312 [========================>.....] - ETA: 1s - loss: 2.1895 - accuracy: 0.2380#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015262/312 [========================>.....] - ETA: 1s - loss: 2.1872 - accuracy: 0.2385#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015264/312 [========================>.....] - ETA: 1s - loss: 2.1848 - accuracy: 0.2390#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015266/312 [========================>.....] - ETA: 1s - loss: 2.1826 - accuracy: 0.2395#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015268/312 [========================>.....] - ETA: 1s - loss: 2.1803 - accuracy: 0.2401#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015270/312 [========================>.....] - ETA: 1s - loss: 2.1780 - accuracy: 0.2406#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015272/312 [=========================>....] - ETA: 1s - loss: 2.1758 - accuracy: 0.2411#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015274/312 [=========================>....] - ETA: 1s - loss: 2.1736 - accuracy: 0.2416#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015276/312 [=========================>....] - ETA: 1s - loss: 2.1714 - accuracy: 0.2421#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015278/312 [=========================>....] - ETA: 1s - loss: 2.1693 - accuracy: 0.2426#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015280/312 [=========================>....] - ETA: 1s - loss: 2.1671 - accuracy: 0.2431#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015282/312 [==========================>...] - ETA: 0s - loss: 2.1650 - accuracy: 0.2436#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015284/312 [==========================>...] - ETA: 0s - loss: 2.1629 - accuracy: 0.2441#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015286/312 [==========================>...] - ETA: 0s - loss: 2.1608 - accuracy: 0.2446#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015288/312 [==========================>...] - ETA: 0s - loss: 2.1587 - accuracy: 0.2451#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015290/312 [==========================>...] - ETA: 0s - loss: 2.1566 - accuracy: 0.2456#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015292/312 [===========================>..] - ETA: 0s - loss: 2.1545 - accuracy: 0.2461#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015294/312 [===========================>..] - ETA: 0s - loss: 2.1525 - accuracy: 0.2465#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015296/312 [===========================>..] - ETA: 0s - loss: 2.1505 - accuracy: 0.2470#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015298/312 [===========================>..] - ETA: 0s - loss: 2.1485 - accuracy: 0.2475#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015300/312 [===========================>..] - ETA: 0s - loss: 2.1465 - accuracy: 0.2480#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015302/312 [============================>.] - ETA: 0s - loss: 2.1445 - accuracy: 0.2485#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015304/312 [============================>.] - ETA: 0s - loss: 2.1425 - accuracy: 0.2489#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015306/312 [============================>.] - ETA: 0s - loss: 2.1405 - accuracy: 0.2494#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015308/312 [============================>.] - ETA: 0s - loss: 2.1386 - accuracy: 0.2499#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015310/312 [============================>.] - ETA: 0s - loss: 2.1366 - accuracy: 0.2504#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015312/312 [==============================] - ETA: 0s - loss: 2.1347 - accuracy: 0.2508#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015312/312 [==============================] - 23s 36ms/step - loss: 2.1338 - accuracy: 0.2511 - val_loss: 1.6756 - val_accuracy: 0.4084[0m
[34mEpoch 2/5[0m
[34m#015 1/312 [..............................] - ETA: 8s - loss: 1.5448 - accuracy: 0.4531#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 3/312 [..............................] - ETA: 8s - loss: 1.5196 - accuracy: 0.4449#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 5/312 [..............................] - ETA: 8s - loss: 1.5302 - accuracy: 0.4390#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 7/312 [..............................] - ETA: 8s - loss: 1.5383 - accuracy: 0.4368#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 9/312 [..............................] - ETA: 8s - loss: 1.5405 - accuracy: 0.4357#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 11/312 [>.............................] - ETA: 8s - loss: 1.5429 - accuracy: 0.4337#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 13/312 [>.............................] - ETA: 8s - loss: 1.5443 - accuracy: 0.4326#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 15/312 [>.............................] - ETA: 8s - loss: 1.5469 - accuracy: 0.4309#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 17/312 [>.............................] - ETA: 8s - loss: 1.5479 - accuracy: 0.4302#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 19/312 [>.............................] - ETA: 8s - loss: 1.5485 - accuracy: 0.4298#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 21/312 [=>............................] - ETA: 8s - loss: 1.5486 - accuracy: 0.4293#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 23/312 [=>............................] - ETA: 8s - loss: 1.5479 - accuracy: 0.4290#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 25/312 [=>............................] - ETA: 8s - loss: 1.5468 - accuracy: 0.4288#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 27/312 [=>............................] - ETA: 8s - loss: 1.5458 - accuracy: 0.4288#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 29/312 [=>............................] - ETA: 8s - loss: 1.5446 - accuracy: 0.4292#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 31/312 [=>............................] - ETA: 8s - loss: 1.5431 - accuracy: 0.4296#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 33/312 [==>...........................] - ETA: 8s - loss: 1.5417 - accuracy: 0.4301#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 35/312 [==>...........................] - ETA: 8s - loss: 1.5404 - accuracy: 0.4305#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 37/312 [==>...........................] - ETA: 7s - loss: 1.5391 - accuracy: 0.4309#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 39/312 [==>...........................] - ETA: 7s - loss: 1.5379 - accuracy: 0.4313#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 41/312 [==>...........................] - ETA: 7s - loss: 1.5367 - accuracy: 0.4316#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 43/312 [===>..........................] - ETA: 7s - loss: 1.5359 - accuracy: 0.4319#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 45/312 [===>..........................] - ETA: 7s - loss: 1.5351 - accuracy: 0.4322#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 47/312 [===>..........................] - ETA: 7s - loss: 1.5342 - accuracy: 0.4325#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 49/312 [===>..........................] - ETA: 7s - loss: 1.5336 - accuracy: 0.4328#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 51/312 [===>..........................] - ETA: 7s - loss: 1.5330 - accuracy: 0.4331#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 53/312 [====>.........................] - ETA: 7s - loss: 1.5324 - accuracy: 0.4333#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 55/312 [====>.........................] - ETA: 7s - loss: 1.5318 - accuracy: 0.4335#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 57/312 [====>.........................] - ETA: 7s - loss: 1.5313 - accuracy: 0.4338#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 59/312 [====>.........................] - ETA: 7s - loss: 1.5307 - accuracy: 0.4340#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 61/312 [====>.........................] - ETA: 7s - loss: 1.5302 - accuracy: 0.4342#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 63/312 [=====>........................] - ETA: 7s - loss: 1.5297 - accuracy: 0.4344#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 65/312 [=====>........................] - ETA: 7s - loss: 1.5292 - accuracy: 0.4346#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 67/312 [=====>........................] - ETA: 7s - loss: 1.5288 - accuracy: 0.4347#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 69/312 [=====>........................] - ETA: 7s - loss: 1.5284 - accuracy: 0.4349#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 71/312 [=====>........................] - ETA: 7s - loss: 1.5280 - accuracy: 0.4351#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 73/312 [======>.......................] - ETA: 6s - loss: 1.5277 - accuracy: 0.4353#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 75/312 [======>.......................] - ETA: 6s - loss: 1.5273 - accuracy: 0.4355#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 77/312 [======>.......................] - ETA: 6s - loss: 1.5269 - accuracy: 0.4357#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 79/312 [======>.......................] - ETA: 6s - loss: 1.5265 - accuracy: 0.4359#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 81/312 [======>.......................] - ETA: 6s - loss: 1.5261 - accuracy: 0.4361#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 83/312 [======>.......................] - ETA: 6s - loss: 1.5256 - accuracy: 0.4363#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 85/312 [=======>......................] - ETA: 6s - loss: 1.5251 - accuracy: 0.4366#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 87/312 [=======>......................] - ETA: 6s - loss: 1.5247 - accuracy: 0.4368#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 89/312 [=======>......................] - ETA: 6s - loss: 1.5242 - accuracy: 0.4370#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 91/312 [=======>......................] - ETA: 6s - loss: 1.5238 - accuracy: 0.4373#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 93/312 [=======>......................] - ETA: 6s - loss: 1.5234 - accuracy: 0.4375#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 95/312 [========>.....................] - ETA: 6s - loss: 1.5231 - accuracy: 0.4377#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 97/312 [========>.....................] - ETA: 6s - loss: 1.5227 - accuracy: 0.4379#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 99/312 [========>.....................] - ETA: 6s - loss: 1.5223 - accuracy: 0.4382#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015101/312 [========>.....................] - ETA: 6s - loss: 1.5218 - accuracy: 0.4384#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015103/312 [========>.....................] - ETA: 6s - loss: 1.5214 - accuracy: 0.4386#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015105/312 [=========>....................] - ETA: 6s - loss: 1.5210 - accuracy: 0.4389#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015107/312 [=========>....................] - ETA: 5s - loss: 1.5206 - accuracy: 0.4391#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015109/312 [=========>....................] - ETA: 5s - loss: 1.5201 - accuracy: 0.4393#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015111/312 [=========>....................] - ETA: 5s - loss: 1.5197 - accuracy: 0.4396#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015113/312 [=========>....................] - ETA: 5s - loss: 1.5192 - accuracy: 0.4398#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015115/312 [==========>...................] - ETA: 5s - loss: 1.5188 - accuracy: 0.4401#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015117/312 [==========>...................] - ETA: 5s - loss: 1.5183 - accuracy: 0.4403#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015119/312 [==========>...................] - ETA: 5s - loss: 1.5178 - accuracy: 0.4406#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015121/312 [==========>...................] - ETA: 5s - loss: 1.5174 - accuracy: 0.4408#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015123/312 [==========>...................] - ETA: 5s - loss: 1.5169 - accuracy: 0.4410#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015125/312 [===========>..................] - ETA: 5s - loss: 1.5164 - accuracy: 0.4413#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015127/312 [===========>..................] - ETA: 5s - loss: 1.5159 - accuracy: 0.4415#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015129/312 [===========>..................] - ETA: 5s - loss: 1.5154 - accuracy: 0.4417#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015131/312 [===========>..................] - ETA: 5s - loss: 1.5150 - accuracy: 0.4420#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015133/312 [===========>..................] - ETA: 5s - loss: 1.5145 - accuracy: 0.4422#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015135/312 [===========>..................] - ETA: 5s - loss: 1.5140 - accuracy: 0.4424#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015137/312 [============>.................] - ETA: 5s - loss: 1.5136 - accuracy: 0.4426#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015139/312 [============>.................] - ETA: 5s - loss: 1.5131 - accuracy: 0.4428#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015141/312 [============>.................] - ETA: 4s - loss: 1.5127 - accuracy: 0.4430#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015143/312 [============>.................] - ETA: 4s - loss: 1.5122 - accuracy: 0.4432#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015145/312 [============>.................] - ETA: 4s - loss: 1.5118 - accuracy: 0.4434#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015147/312 [=============>................] - ETA: 4s - loss: 1.5114 - accuracy: 0.4436#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015149/312 [=============>................] - ETA: 4s - loss: 1.5110 - accuracy: 0.4438#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015151/312 [=============>................] - ETA: 4s - loss: 1.5105 - accuracy: 0.4440#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015153/312 [=============>................] - ETA: 4s - loss: 1.5101 - accuracy: 0.4442#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015155/312 [=============>................] - ETA: 4s - loss: 1.5096 - accuracy: 0.4444#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015157/312 [==============>...............] - ETA: 4s - loss: 1.5091 - accuracy: 0.4446#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015159/312 [==============>...............] - ETA: 4s - loss: 1.5087 - accuracy: 0.4448#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015161/312 [==============>...............] - ETA: 4s - loss: 1.5082 - accuracy: 0.4450#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015163/312 [==============>...............] - ETA: 4s - loss: 1.5078 - accuracy: 0.4452#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015165/312 [==============>...............] - ETA: 4s - loss: 1.5073 - accuracy: 0.4454#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015167/312 [===============>..............] - ETA: 4s - loss: 1.5069 - accuracy: 0.4456#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015169/312 [===============>..............] - ETA: 4s - loss: 1.5064 - accuracy: 0.4458#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015171/312 [===============>..............] - ETA: 4s - loss: 1.5060 - accuracy: 0.4460#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015173/312 [===============>..............] - ETA: 4s - loss: 1.5056 - accuracy: 0.4462#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015175/312 [===============>..............] - ETA: 3s - loss: 1.5051 - accuracy: 0.4464#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015177/312 [================>.............] - ETA: 3s - loss: 1.5047 - accuracy: 0.4466#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015179/312 [================>.............] - ETA: 3s - loss: 1.5042 - accuracy: 0.4468#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015181/312 [================>.............] - ETA: 3s - loss: 1.5038 - accuracy: 0.4470#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015183/312 [================>.............] - ETA: 3s - loss: 1.5033 - accuracy: 0.4472#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015185/312 [================>.............] - ETA: 3s - loss: 1.5029 - accuracy: 0.4474#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015187/312 [================>.............] - ETA: 3s - loss: 1.5025 - accuracy: 0.4476#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015189/312 [=================>............] - ETA: 3s - loss: 1.5020 - accuracy: 0.4477#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015191/312 [=================>............] - ETA: 3s - loss: 1.5016 - accuracy: 0.4479#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015193/312 [=================>............] - ETA: 3s - loss: 1.5012 - accuracy: 0.4481#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010[0m
[34m#010#010#010#010#010#010#010#010#010#015195/312 [=================>............] - ETA: 3s - loss: 1.5008 - accuracy: 0.4483#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015197/312 [=================>............] - ETA: 3s - loss: 1.5004 - accuracy: 0.4485#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015199/312 [==================>...........] - ETA: 3s - loss: 1.5000 - accuracy: 0.4487#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015201/312 [==================>...........] - ETA: 3s - loss: 1.4995 - accuracy: 0.4489#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015203/312 [==================>...........] - ETA: 3s - loss: 1.4991 - accuracy: 0.4491#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015205/312 [==================>...........] - ETA: 3s - loss: 1.4987 - accuracy: 0.4493#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015207/312 [==================>...........] - ETA: 3s - loss: 1.4983 - accuracy: 0.4495#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015209/312 [===================>..........] - ETA: 2s - loss: 1.4979 - accuracy: 0.4497#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015211/312 [===================>..........] - ETA: 2s - loss: 1.4975 - accuracy: 0.4499#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015213/312 [===================>..........] - ETA: 2s - loss: 1.4971 - accuracy: 0.4500#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015215/312 [===================>..........] - ETA: 2s - loss: 1.4967 - accuracy: 0.4502#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015217/312 [===================>..........] - ETA: 2s - loss: 1.4963 - accuracy: 0.4504#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015219/312 [====================>.........] - ETA: 2s - loss: 1.4959 - accuracy: 0.4506#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015221/312 [====================>.........] - ETA: 2s - loss: 1.4955 - accuracy: 0.4508#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015223/312 [====================>.........] - ETA: 2s - loss: 1.4950 - accuracy: 0.4510#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015225/312 [====================>.........] - ETA: 2s - loss: 1.4946 - accuracy: 0.4512#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015227/312 [====================>.........] - ETA: 2s - loss: 1.4942 - accuracy: 0.4514#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015229/312 [=====================>........] - ETA: 2s - loss: 1.4938 - accuracy: 0.4516#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015231/312 [=====================>........] - ETA: 2s - loss: 1.4934 - accuracy: 0.4518#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015233/312 [=====================>........] - ETA: 2s - loss: 1.4929 - accuracy: 0.4520#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015235/312 [=====================>........] - ETA: 2s - loss: 1.4925 - accuracy: 0.4522#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015237/312 [=====================>........] - ETA: 2s - loss: 1.4921 - accuracy: 0.4524#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015239/312 [=====================>........] - ETA: 2s - loss: 1.4917 - accuracy: 0.4526#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015241/312 [======================>.......] - ETA: 2s - loss: 1.4913 - accuracy: 0.4527#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015243/312 [======================>.......] - ETA: 2s - loss: 1.4908 - accuracy: 0.4529#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015245/312 [======================>.......] - ETA: 1s - loss: 1.4904 - accuracy: 0.4531#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015247/312 [======================>.......] - ETA: 1s - loss: 1.4900 - accuracy: 0.4533#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015249/312 [======================>.......] - ETA: 1s - loss: 1.4896 - accuracy: 0.4535#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015251/312 [=======================>......] - ETA: 1s - loss: 1.4893 - accuracy: 0.4536#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015253/312 [=======================>......] - ETA: 1s - loss: 1.4889 - accuracy: 0.4538#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015255/312 [=======================>......] - ETA: 1s - loss: 1.4885 - accuracy: 0.4540#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015257/312 [=======================>......] - ETA: 1s - loss: 1.4881 - accuracy: 0.4542#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015259/312 [=======================>......] - ETA: 1s - loss: 1.4878 - accuracy: 0.4543#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015261/312 [========================>.....] - ETA: 1s - loss: 1.4874 - accuracy: 0.4545#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015263/312 [========================>.....] - ETA: 1s - loss: 1.4871 - accuracy: 0.4546#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015265/312 [========================>.....] - ETA: 1s - loss: 1.4867 - accuracy: 0.4548#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015267/312 [========================>.....] - ETA: 1s - loss: 1.4863 - accuracy: 0.4550#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015269/312 [========================>.....] - ETA: 1s - loss: 1.4860 - accuracy: 0.4551#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015271/312 [=========================>....] - ETA: 1s - loss: 1.4856 - accuracy: 0.4553#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015273/312 [=========================>....] - ETA: 1s - loss: 1.4852 - accuracy: 0.4555#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015275/312 [=========================>....] - ETA: 1s - loss: 1.4848 - accuracy: 0.4556#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015277/312 [=========================>....] - ETA: 1s - loss: 1.4845 - accuracy: 0.4558#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015279/312 [=========================>....] - ETA: 0s - loss: 1.4841 - accuracy: 0.4560#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015281/312 [==========================>...] - ETA: 0s - loss: 1.4837 - accuracy: 0.4561#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015283/312 [==========================>...] - ETA: 0s - loss: 1.4833 - accuracy: 0.4563#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015285/312 [==========================>...] - ETA: 0s - loss: 1.4829 - accuracy: 0.4565#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015287/312 [==========================>...] - ETA: 0s - loss: 1.4825 - accuracy: 0.4567#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015289/312 [==========================>...] - ETA: 0s - loss: 1.4821 - accuracy: 0.4568#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015291/312 [==========================>...] - ETA: 0s - loss: 1.4818 - accuracy: 0.4570#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015293/312 [===========================>..] - ETA: 0s - loss: 1.4814 - accuracy: 0.4572#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015295/312 [===========================>..] - ETA: 0s - loss: 1.4810 - accuracy: 0.4573#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015297/312 [===========================>..] - ETA: 0s - loss: 1.4806 - accuracy: 0.4575#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015299/312 [===========================>..] - ETA: 0s - loss: 1.4802 - accuracy: 0.4577#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015301/312 [===========================>..] - ETA: 0s - loss: 1.4798 - accuracy: 0.4578#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015303/312 [============================>.] - ETA: 0s - loss: 1.4794 - accuracy: 0.4580#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015305/312 [============================>.] - ETA: 0s - loss: 1.4790 - accuracy: 0.4582#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015307/312 [============================>.] - ETA: 0s - loss: 1.4786 - accuracy: 0.4583#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015309/312 [============================>.] - ETA: 0s - loss: 1.4782 - accuracy: 0.4585#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015311/312 [============================>.] - ETA: 0s - loss: 1.4778 - accuracy: 0.4587#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015312/312 [==============================] - 10s 31ms/step - loss: 1.4774 - accuracy: 0.4588 - val_loss: 1.4685 - val_accuracy: 0.4654[0m
[34mEpoch 3/5[0m
[34m#015 1/312 [..............................] - ETA: 9s - loss: 1.5111 - accuracy: 0.4453#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 3/312 [..............................] - ETA: 8s - loss: 1.3716 - accuracy: 0.5052#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 5/312 [..............................] - ETA: 8s - loss: 1.3366 - accuracy: 0.5173#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 7/312 [..............................] - ETA: 8s - loss: 1.3296 - accuracy: 0.5200#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 9/312 [..............................] - ETA: 8s - loss: 1.3249 - accuracy: 0.5226#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 11/312 [>.............................] - ETA: 8s - loss: 1.3181 - accuracy: 0.5257#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 13/312 [>.............................] - ETA: 8s - loss: 1.3125 - accuracy: 0.5273#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 15/312 [>.............................] - ETA: 8s - loss: 1.3086 - accuracy: 0.5280#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 17/312 [>.............................] - ETA: 8s - loss: 1.3068 - accuracy: 0.5284#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 19/312 [>.............................] - ETA: 8s - loss: 1.3045 - accuracy: 0.5292#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 21/312 [=>............................] - ETA: 8s - loss: 1.3030 - accuracy: 0.5298#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 23/312 [=>............................] - ETA: 8s - loss: 1.3009 - accuracy: 0.5306#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 25/312 [=>............................] - ETA: 8s - loss: 1.2990 - accuracy: 0.5316#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 27/312 [=>............................] - ETA: 8s - loss: 1.2972 - accuracy: 0.5326#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 29/312 [=>............................] - ETA: 8s - loss: 1.2955 - accuracy: 0.5336#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 31/312 [=>............................] - ETA: 8s - loss: 1.2938 - accuracy: 0.5347#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 33/312 [==>...........................] - ETA: 8s - loss: 1.2927 - accuracy: 0.5353#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 35/312 [==>...........................] - ETA: 8s - loss: 1.2918 - accuracy: 0.5358#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 37/312 [==>...........................] - ETA: 8s - loss: 1.2912 - accuracy: 0.5361#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 39/312 [==>...........................] - ETA: 7s - loss: 1.2906 - accuracy: 0.5364#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 41/312 [==>...........................] - ETA: 7s - loss: 1.2899 - accuracy: 0.5367#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 43/312 [===>..........................] - ETA: 7s - loss: 1.2893 - accuracy: 0.5370#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 45/312 [===>..........................] - ETA: 7s - loss: 1.2888 - accuracy: 0.5372#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 47/312 [===>..........................] - ETA: 7s - loss: 1.2883 - accuracy: 0.5373#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 49/312 [===>..........................] - ETA: 7s - loss: 1.2879 - accuracy: 0.5374#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 51/312 [===>..........................] - ETA: 7s - loss: 1.2877 - accuracy: 0.5374#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 53/312 [====>.........................] - ETA: 7s - loss: 1.2873 - accuracy: 0.5375#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 55/312 [====>.........................] - ETA: 7s - loss: 1.2869 - accuracy: 0.5375#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 57/312 [====>.........................] - ETA: 7s - loss: 1.2865 - accuracy: 0.5375#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 59/312 [====>.........................] - ETA: 7s - loss: 1.2859 - accuracy: 0.5376#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 61/312 [====>.........................] - ETA: 7s - loss: 1.2852 - accuracy: 0.5377#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 63/312 [=====>........................] - ETA: 7s - loss: 1.2845 - accuracy: 0.5378#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 65/312 [=====>........................] - ETA: 7s - loss: 1.2837 - accuracy: 0.5379#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 67/312 [=====>........................] - ETA: 7s - loss: 1.2831 - accuracy: 0.5380#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 69/312 [=====>........................] - ETA: 7s - loss: 1.2824 - accuracy: 0.5382#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 71/312 [=====>........................] - ETA: 7s - loss: 1.2817 - accuracy: 0.5384#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 73/312 [======>.......................] - ETA: 6s - loss: 1.2812 - accuracy: 0.5385#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 75/312 [======>.......................] - ETA: 6s - loss: 1.2808 - accuracy: 0.5386#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 77/312 [======>.......................] - ETA: 6s - loss: 1.2804 - accuracy: 0.5387#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 79/312 [======>.......................] - ETA: 6s - loss: 1.2799 - accuracy: 0.5389#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 81/312 [======>.......................] - ETA: 6s - loss: 1.2796 - accuracy: 0.5390#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 83/312 [======>.......................] - ETA: 6s - loss: 1.2792 - accuracy: 0.5391#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 85/312 [=======>......................] - ETA: 6s - loss: 1.2789 - accuracy: 0.5392#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 87/312 [=======>......................] - ETA: 6s - loss: 1.2785 - accuracy: 0.5393#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 89/312 [=======>......................] - ETA: 6s - loss: 1.2782 - accuracy: 0.5394#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 91/312 [=======>......................] - ETA: 6s - loss: 1.2778 - accuracy: 0.5395#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 93/312 [=======>......................] - ETA: 6s - loss: 1.2775 - accuracy: 0.5396#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 95/312 [========>.....................] - ETA: 6s - loss: 1.2772 - accuracy: 0.5397#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 97/312 [========>.....................] - ETA: 6s - loss: 1.2769 - accuracy: 0.5398#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 99/312 [========>.....................] - ETA: 6s - loss: 1.2765 - accuracy: 0.5399#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015101/312 [========>.....................] - ETA: 6s - loss: 1.2762 - accuracy: 0.5400#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015103/312 [========>.....................] - ETA: 6s - loss: 1.2759 - accuracy: 0.5400#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015105/312 [=========>....................] - ETA: 6s - loss: 1.2755 - accuracy: 0.5401#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015107/312 [=========>....................] - ETA: 5s - loss: 1.2752 - accuracy: 0.5402#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015109/312 [=========>....................] - ETA: 5s - loss: 1.2749 - accuracy: 0.5402#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015111/312 [=========>....................] - ETA: 5s - loss: 1.2746 - accuracy: 0.5403#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015113/312 [=========>....................] - ETA: 5s - loss: 1.2743 - accuracy: 0.5404#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015115/312 [==========>...................] - ETA: 5s - loss: 1.2739 - accuracy: 0.5404#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015117/312 [==========>...................] - ETA: 5s - loss: 1.2736 - accuracy: 0.5405#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015119/312 [==========>...................] - ETA: 5s - loss: 1.2733 - accuracy: 0.5406#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015121/312 [==========>...................] - ETA: 5s - loss: 1.2730 - accuracy: 0.5406#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015123/312 [==========>...................] - ETA: 5s - loss: 1.2727 - accuracy: 0.5407#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015125/312 [===========>..................] - ETA: 5s - loss: 1.2724 - accuracy: 0.5407#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015127/312 [===========>..................] - ETA: 5s - loss: 1.2721 - accuracy: 0.5408#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015129/312 [===========>..................] - ETA: 5s - loss: 1.2718 - accuracy: 0.5409#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015131/312 [===========>..................] - ETA: 5s - loss: 1.2716 - accuracy: 0.5409#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015133/312 [===========>..................] - ETA: 5s - loss: 1.2713 - accuracy: 0.5410#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015135/312 [===========>..................] - ETA: 5s - loss: 1.2711 - accuracy: 0.5411#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015137/312 [============>.................] - ETA: 5s - loss: 1.2709 - accuracy: 0.5411#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015139/312 [============>.................] - ETA: 5s - loss: 1.2707 - accuracy: 0.5412#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015141/312 [============>.................] - ETA: 4s - loss: 1.2704 - accuracy: 0.5413#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015143/312 [============>.................] - ETA: 4s - loss: 1.2702 - accuracy: 0.5413#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015145/312 [============>.................] - ETA: 4s - loss: 1.2701 - accuracy: 0.5414#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015147/312 [=============>................] - ETA: 4s - loss: 1.2699 - accuracy: 0.5414#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015149/312 [=============>................] - ETA: 4s - loss: 1.2697 - accuracy: 0.5415#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015151/312 [=============>................] - ETA: 4s - loss: 1.2695 - accuracy: 0.5416#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015153/312 [=============>................] - ETA: 4s - loss: 1.2693 - accuracy: 0.5416#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015155/312 [=============>................] - ETA: 4s - loss: 1.2692 - accuracy: 0.5417#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015157/312 [==============>...............] - ETA: 4s - loss: 1.2690 - accuracy: 0.5418#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015159/312 [==============>...............] - ETA: 4s - loss: 1.2688 - accuracy: 0.5418#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015161/312 [==============>...............] - ETA: 4s - loss: 1.2687 - accuracy: 0.5419#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015163/312 [==============>...............] - ETA: 4s - loss: 1.2685 - accuracy: 0.5419#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015165/312 [==============>...............] - ETA: 4s - loss: 1.2684 - accuracy: 0.5420#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015167/312 [===============>..............] - ETA: 4s - loss: 1.2683 - accuracy: 0.5420#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015169/312 [===============>..............] - ETA: 4s - loss: 1.2682 - accuracy: 0.5421#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015171/312 [===============>..............] - ETA: 4s - loss: 1.2680 - accuracy: 0.5421#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015173/312 [===============>..............] - ETA: 4s - loss: 1.2679 - accuracy: 0.5422#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015175/312 [===============>..............] - ETA: 3s - loss: 1.2677 - accuracy: 0.5422#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015177/312 [================>.............] - ETA: 3s - loss: 1.2676 - accuracy: 0.5423#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015179/312 [================>.............] - ETA: 3s - loss: 1.2674 - accuracy: 0.5424#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015181/312 [================>.............] - ETA: 3s - loss: 1.2673 - accuracy: 0.5424#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015183/312 [================>.............] - ETA: 3s - loss: 1.2672 - accuracy: 0.5425#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015185/312 [================>.............] - ETA: 3s - loss: 1.2670 - accuracy: 0.5425#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015187/312 [================>.............] - ETA: 3s - loss: 1.2669 - accuracy: 0.5426#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015189/312 [=================>............] - ETA: 3s - loss: 1.2667 - accuracy: 0.5427#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015191/312 [=================>............] - ETA: 3s - loss: 1.2666 - accuracy: 0.5427#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015193/312 [=================>............] - ETA: 3s - loss: 1.2664 - accuracy: 0.5428#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010[0m
[34m#010#010#010#010#010#010#010#010#010#015195/312 [=================>............] - ETA: 3s - loss: 1.2663 - accuracy: 0.5429#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015197/312 [=================>............] - ETA: 3s - loss: 1.2661 - accuracy: 0.5430#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015199/312 [==================>...........] - ETA: 3s - loss: 1.2659 - accuracy: 0.5430#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015201/312 [==================>...........] - ETA: 3s - loss: 1.2658 - accuracy: 0.5431#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015203/312 [==================>...........] - ETA: 3s - loss: 1.2656 - accuracy: 0.5432#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015205/312 [==================>...........] - ETA: 3s - loss: 1.2654 - accuracy: 0.5433#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015207/312 [==================>...........] - ETA: 3s - loss: 1.2653 - accuracy: 0.5433#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015209/312 [===================>..........] - ETA: 2s - loss: 1.2651 - accuracy: 0.5434#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015211/312 [===================>..........] - ETA: 2s - loss: 1.2650 - accuracy: 0.5435#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015213/312 [===================>..........] - ETA: 2s - loss: 1.2648 - accuracy: 0.5436#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015215/312 [===================>..........] - ETA: 2s - loss: 1.2646 - accuracy: 0.5436#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015217/312 [===================>..........] - ETA: 2s - loss: 1.2644 - accuracy: 0.5437#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015219/312 [====================>.........] - ETA: 2s - loss: 1.2642 - accuracy: 0.5438#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015221/312 [====================>.........] - ETA: 2s - loss: 1.2641 - accuracy: 0.5439#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015223/312 [====================>.........] - ETA: 2s - loss: 1.2639 - accuracy: 0.5440#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015225/312 [====================>.........] - ETA: 2s - loss: 1.2637 - accuracy: 0.5441#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015227/312 [====================>.........] - ETA: 2s - loss: 1.2635 - accuracy: 0.5441#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015229/312 [=====================>........] - ETA: 2s - loss: 1.2633 - accuracy: 0.5442#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015231/312 [=====================>........] - ETA: 2s - loss: 1.2631 - accuracy: 0.5443#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015233/312 [=====================>........] - ETA: 2s - loss: 1.2629 - accuracy: 0.5444#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015235/312 [=====================>........] - ETA: 2s - loss: 1.2627 - accuracy: 0.5445#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015237/312 [=====================>........] - ETA: 2s - loss: 1.2625 - accuracy: 0.5446#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015239/312 [=====================>........] - ETA: 2s - loss: 1.2623 - accuracy: 0.5447#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015241/312 [======================>.......] - ETA: 2s - loss: 1.2621 - accuracy: 0.5447#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015243/312 [======================>.......] - ETA: 2s - loss: 1.2619 - accuracy: 0.5448#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015245/312 [======================>.......] - ETA: 1s - loss: 1.2617 - accuracy: 0.5449#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015247/312 [======================>.......] - ETA: 1s - loss: 1.2615 - accuracy: 0.5450#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015249/312 [======================>.......] - ETA: 1s - loss: 1.2613 - accuracy: 0.5451#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015251/312 [=======================>......] - ETA: 1s - loss: 1.2611 - accuracy: 0.5451#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015253/312 [=======================>......] - ETA: 1s - loss: 1.2609 - accuracy: 0.5452#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015255/312 [=======================>......] - ETA: 1s - loss: 1.2608 - accuracy: 0.5453#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015257/312 [=======================>......] - ETA: 1s - loss: 1.2606 - accuracy: 0.5454#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015259/312 [=======================>......] - ETA: 1s - loss: 1.2604 - accuracy: 0.5454#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015261/312 [========================>.....] - ETA: 1s - loss: 1.2602 - accuracy: 0.5455#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015263/312 [========================>.....] - ETA: 1s - loss: 1.2600 - accuracy: 0.5456#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015265/312 [========================>.....] - ETA: 1s - loss: 1.2598 - accuracy: 0.5457#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015267/312 [========================>.....] - ETA: 1s - loss: 1.2596 - accuracy: 0.5457#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015269/312 [========================>.....] - ETA: 1s - loss: 1.2594 - accuracy: 0.5458#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015271/312 [=========================>....] - ETA: 1s - loss: 1.2592 - accuracy: 0.5459#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015273/312 [=========================>....] - ETA: 1s - loss: 1.2590 - accuracy: 0.5460#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015275/312 [=========================>....] - ETA: 1s - loss: 1.2588 - accuracy: 0.5461#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015277/312 [=========================>....] - ETA: 1s - loss: 1.2586 - accuracy: 0.5461#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015279/312 [=========================>....] - ETA: 0s - loss: 1.2584 - accuracy: 0.5462#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015281/312 [==========================>...] - ETA: 0s - loss: 1.2583 - accuracy: 0.5463#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015283/312 [==========================>...] - ETA: 0s - loss: 1.2581 - accuracy: 0.5463#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015285/312 [==========================>...] - ETA: 0s - loss: 1.2579 - accuracy: 0.5464#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015287/312 [==========================>...] - ETA: 0s - loss: 1.2577 - accuracy: 0.5465#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015289/312 [==========================>...] - ETA: 0s - loss: 1.2575 - accuracy: 0.5466#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015291/312 [==========================>...] - ETA: 0s - loss: 1.2574 - accuracy: 0.5466#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015293/312 [===========================>..] - ETA: 0s - loss: 1.2572 - accuracy: 0.5467#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015295/312 [===========================>..] - ETA: 0s - loss: 1.2570 - accuracy: 0.5468#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015297/312 [===========================>..] - ETA: 0s - loss: 1.2568 - accuracy: 0.5468#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015299/312 [===========================>..] - ETA: 0s - loss: 1.2567 - accuracy: 0.5469#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015301/312 [===========================>..] - ETA: 0s - loss: 1.2565 - accuracy: 0.5470#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015303/312 [============================>.] - ETA: 0s - loss: 1.2563 - accuracy: 0.5470#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015305/312 [============================>.] - ETA: 0s - loss: 1.2561 - accuracy: 0.5471#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015307/312 [============================>.] - ETA: 0s - loss: 1.2560 - accuracy: 0.5472#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015309/312 [============================>.] - ETA: 0s - loss: 1.2558 - accuracy: 0.5472#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015311/312 [============================>.] - ETA: 0s - loss: 1.2556 - accuracy: 0.5473#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015312/312 [==============================] - 10s 32ms/step - loss: 1.2554 - accuracy: 0.5474 - val_loss: 1.1826 - val_accuracy: 0.5735[0m
[34mEpoch 4/5[0m
[34m#015 1/312 [..............................] - ETA: 9s - loss: 1.2226 - accuracy: 0.6016#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 3/312 [..............................] - ETA: 9s - loss: 1.1838 - accuracy: 0.5929#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 5/312 [..............................] - ETA: 8s - loss: 1.1707 - accuracy: 0.5983#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 7/312 [..............................] - ETA: 8s - loss: 1.1572 - accuracy: 0.6042#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 9/312 [..............................] - ETA: 8s - loss: 1.1505 - accuracy: 0.6048#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 11/312 [>.............................] - ETA: 8s - loss: 1.1437 - accuracy: 0.6058#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 13/312 [>.............................] - ETA: 8s - loss: 1.1407 - accuracy: 0.6057#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 15/312 [>.............................] - ETA: 8s - loss: 1.1392 - accuracy: 0.6056#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 17/312 [>.............................] - ETA: 8s - loss: 1.1393 - accuracy: 0.6049#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 19/312 [>.............................] - ETA: 8s - loss: 1.1392 - accuracy: 0.6042#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 21/312 [=>............................] - ETA: 8s - loss: 1.1388 - accuracy: 0.6037#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 23/312 [=>............................] - ETA: 8s - loss: 1.1381 - accuracy: 0.6034#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 25/312 [=>............................] - ETA: 8s - loss: 1.1372 - accuracy: 0.6034#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 27/312 [=>............................] - ETA: 8s - loss: 1.1361 - accuracy: 0.6036#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 29/312 [=>............................] - ETA: 8s - loss: 1.1360 - accuracy: 0.6035#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 31/312 [=>............................] - ETA: 8s - loss: 1.1362 - accuracy: 0.6032#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 33/312 [==>...........................] - ETA: 8s - loss: 1.1364 - accuracy: 0.6028#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 35/312 [==>...........................] - ETA: 8s - loss: 1.1369 - accuracy: 0.6022#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 37/312 [==>...........................] - ETA: 7s - loss: 1.1374 - accuracy: 0.6016#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 39/312 [==>...........................] - ETA: 7s - loss: 1.1376 - accuracy: 0.6011#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 41/312 [==>...........................] - ETA: 7s - loss: 1.1376 - accuracy: 0.6007#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 43/312 [===>..........................] - ETA: 7s - loss: 1.1375 - accuracy: 0.6003#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 45/312 [===>..........................] - ETA: 7s - loss: 1.1374 - accuracy: 0.6000#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 47/312 [===>..........................] - ETA: 7s - loss: 1.1373 - accuracy: 0.5996#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 49/312 [===>..........................] - ETA: 7s - loss: 1.1374 - accuracy: 0.5993#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 51/312 [===>..........................] - ETA: 7s - loss: 1.1376 - accuracy: 0.5988#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 53/312 [====>.........................] - ETA: 7s - loss: 1.1378 - accuracy: 0.5984#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 55/312 [====>.........................] - ETA: 7s - loss: 1.1380 - accuracy: 0.5980#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 57/312 [====>.........................] - ETA: 7s - loss: 1.1383 - accuracy: 0.5975#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 59/312 [====>.........................] - ETA: 7s - loss: 1.1386 - accuracy: 0.5971#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 61/312 [====>.........................] - ETA: 7s - loss: 1.1388 - accuracy: 0.5967#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 63/312 [=====>........................] - ETA: 7s - loss: 1.1390 - accuracy: 0.5964#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 65/312 [=====>........................] - ETA: 7s - loss: 1.1392 - accuracy: 0.5961#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 67/312 [=====>........................] - ETA: 7s - loss: 1.1394 - accuracy: 0.5958#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 69/312 [=====>........................] - ETA: 7s - loss: 1.1396 - accuracy: 0.5955#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 71/312 [=====>........................] - ETA: 7s - loss: 1.1398 - accuracy: 0.5953#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 73/312 [======>.......................] - ETA: 6s - loss: 1.1399 - accuracy: 0.5951#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 75/312 [======>.......................] - ETA: 6s - loss: 1.1399 - accuracy: 0.5949#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 77/312 [======>.......................] - ETA: 6s - loss: 1.1399 - accuracy: 0.5948#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 79/312 [======>.......................] - ETA: 6s - loss: 1.1399 - accuracy: 0.5946#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 81/312 [======>.......................] - ETA: 6s - loss: 1.1399 - accuracy: 0.5945#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 83/312 [======>.......................] - ETA: 6s - loss: 1.1399 - accuracy: 0.5944#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 85/312 [=======>......................] - ETA: 6s - loss: 1.1399 - accuracy: 0.5943#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 87/312 [=======>......................] - ETA: 6s - loss: 1.1400 - accuracy: 0.5942#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 89/312 [=======>......................] - ETA: 6s - loss: 1.1400 - accuracy: 0.5941#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 91/312 [=======>......................] - ETA: 6s - loss: 1.1400 - accuracy: 0.5940#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 93/312 [=======>......................] - ETA: 6s - loss: 1.1399 - accuracy: 0.5940#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 95/312 [========>.....................] - ETA: 6s - loss: 1.1399 - accuracy: 0.5939#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 97/312 [========>.....................] - ETA: 6s - loss: 1.1398 - accuracy: 0.5938#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 99/312 [========>.....................] - ETA: 6s - loss: 1.1398 - accuracy: 0.5938#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015101/312 [========>.....................] - ETA: 6s - loss: 1.1397 - accuracy: 0.5938#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015103/312 [========>.....................] - ETA: 6s - loss: 1.1396 - accuracy: 0.5937#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015105/312 [=========>....................] - ETA: 6s - loss: 1.1396 - accuracy: 0.5937#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015107/312 [=========>....................] - ETA: 5s - loss: 1.1396 - accuracy: 0.5937#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015109/312 [=========>....................] - ETA: 5s - loss: 1.1395 - accuracy: 0.5937#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015111/312 [=========>....................] - ETA: 5s - loss: 1.1394 - accuracy: 0.5936#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015113/312 [=========>....................] - ETA: 5s - loss: 1.1394 - accuracy: 0.5936#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015115/312 [==========>...................] - ETA: 5s - loss: 1.1393 - accuracy: 0.5936#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015117/312 [==========>...................] - ETA: 5s - loss: 1.1393 - accuracy: 0.5936#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015119/312 [==========>...................] - ETA: 5s - loss: 1.1392 - accuracy: 0.5935#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015121/312 [==========>...................] - ETA: 5s - loss: 1.1392 - accuracy: 0.5935#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015123/312 [==========>...................] - ETA: 5s - loss: 1.1391 - accuracy: 0.5935#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015125/312 [===========>..................] - ETA: 5s - loss: 1.1391 - accuracy: 0.5935#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015127/312 [===========>..................] - ETA: 5s - loss: 1.1390 - accuracy: 0.5934#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015129/312 [===========>..................] - ETA: 5s - loss: 1.1390 - accuracy: 0.5934#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015131/312 [===========>..................] - ETA: 5s - loss: 1.1389 - accuracy: 0.5934#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015133/312 [===========>..................] - ETA: 5s - loss: 1.1388 - accuracy: 0.5934#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015135/312 [===========>..................] - ETA: 5s - loss: 1.1388 - accuracy: 0.5934#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015137/312 [============>.................] - ETA: 5s - loss: 1.1387 - accuracy: 0.5934#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015139/312 [============>.................] - ETA: 5s - loss: 1.1386 - accuracy: 0.5934#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015141/312 [============>.................] - ETA: 5s - loss: 1.1385 - accuracy: 0.5934#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015143/312 [============>.................] - ETA: 4s - loss: 1.1384 - accuracy: 0.5934#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015145/312 [============>.................] - ETA: 4s - loss: 1.1383 - accuracy: 0.5934#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015147/312 [=============>................] - ETA: 4s - loss: 1.1382 - accuracy: 0.5934#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015149/312 [=============>................] - ETA: 4s - loss: 1.1380 - accuracy: 0.5934#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015151/312 [=============>................] - ETA: 4s - loss: 1.1379 - accuracy: 0.5934#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015153/312 [=============>................] - ETA: 4s - loss: 1.1378 - accuracy: 0.5934#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015155/312 [=============>................] - ETA: 4s - loss: 1.1377 - accuracy: 0.5934#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015157/312 [==============>...............] - ETA: 4s - loss: 1.1376 - accuracy: 0.5935#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015159/312 [==============>...............] - ETA: 4s - loss: 1.1375 - accuracy: 0.5935#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015161/312 [==============>...............] - ETA: 4s - loss: 1.1374 - accuracy: 0.5935#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015163/312 [==============>...............] - ETA: 4s - loss: 1.1373 - accuracy: 0.5935#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015165/312 [==============>...............] - ETA: 4s - loss: 1.1371 - accuracy: 0.5935#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015167/312 [===============>..............] - ETA: 4s - loss: 1.1370 - accuracy: 0.5936#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015169/312 [===============>..............] - ETA: 4s - loss: 1.1368 - accuracy: 0.5936#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015171/312 [===============>..............] - ETA: 4s - loss: 1.1366 - accuracy: 0.5936#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015173/312 [===============>..............] - ETA: 4s - loss: 1.1365 - accuracy: 0.5936#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015175/312 [===============>..............] - ETA: 4s - loss: 1.1363 - accuracy: 0.5937#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015177/312 [================>.............] - ETA: 3s - loss: 1.1361 - accuracy: 0.5937#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015179/312 [================>.............] - ETA: 3s - loss: 1.1359 - accuracy: 0.5937#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015181/312 [================>.............] - ETA: 3s - loss: 1.1357 - accuracy: 0.5938#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015183/312 [================>.............] - ETA: 3s - loss: 1.1355 - accuracy: 0.5938#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015185/312 [================>.............] - ETA: 3s - loss: 1.1353 - accuracy: 0.5939#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015187/312 [================>.............] - ETA: 3s - loss: 1.1351 - accuracy: 0.5939#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015189/312 [=================>............] - ETA: 3s - loss: 1.1349 - accuracy: 0.5939#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015191/312 [=================>............] - ETA: 3s - loss: 1.1348 - accuracy: 0.5940#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015193/312 [=================>............] - ETA: 3s - loss: 1.1346 - accuracy: 0.5940#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010[0m
[34m#010#010#010#010#010#010#010#010#010#015195/312 [=================>............] - ETA: 3s - loss: 1.1344 - accuracy: 0.5941#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015197/312 [=================>............] - ETA: 3s - loss: 1.1342 - accuracy: 0.5941#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015199/312 [==================>...........] - ETA: 3s - loss: 1.1340 - accuracy: 0.5942#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015201/312 [==================>...........] - ETA: 3s - loss: 1.1338 - accuracy: 0.5942#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015203/312 [==================>...........] - ETA: 3s - loss: 1.1337 - accuracy: 0.5943#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015205/312 [==================>...........] - ETA: 3s - loss: 1.1335 - accuracy: 0.5943#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015207/312 [==================>...........] - ETA: 3s - loss: 1.1333 - accuracy: 0.5944#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015209/312 [===================>..........] - ETA: 3s - loss: 1.1331 - accuracy: 0.5944#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015211/312 [===================>..........] - ETA: 2s - loss: 1.1330 - accuracy: 0.5944#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015213/312 [===================>..........] - ETA: 2s - loss: 1.1328 - accuracy: 0.5945#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015215/312 [===================>..........] - ETA: 2s - loss: 1.1326 - accuracy: 0.5945#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015217/312 [===================>..........] - ETA: 2s - loss: 1.1324 - accuracy: 0.5946#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015219/312 [====================>.........] - ETA: 2s - loss: 1.1322 - accuracy: 0.5946#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015221/312 [====================>.........] - ETA: 2s - loss: 1.1320 - accuracy: 0.5947#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015223/312 [====================>.........] - ETA: 2s - loss: 1.1318 - accuracy: 0.5947#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015225/312 [====================>.........] - ETA: 2s - loss: 1.1317 - accuracy: 0.5948#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015227/312 [====================>.........] - ETA: 2s - loss: 1.1315 - accuracy: 0.5948#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015229/312 [=====================>........] - ETA: 2s - loss: 1.1313 - accuracy: 0.5949#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015231/312 [=====================>........] - ETA: 2s - loss: 1.1311 - accuracy: 0.5949#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015233/312 [=====================>........] - ETA: 2s - loss: 1.1309 - accuracy: 0.5950#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015235/312 [=====================>........] - ETA: 2s - loss: 1.1307 - accuracy: 0.5950#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015237/312 [=====================>........] - ETA: 2s - loss: 1.1305 - accuracy: 0.5951#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015239/312 [=====================>........] - ETA: 2s - loss: 1.1303 - accuracy: 0.5951#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015241/312 [======================>.......] - ETA: 2s - loss: 1.1301 - accuracy: 0.5952#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015243/312 [======================>.......] - ETA: 2s - loss: 1.1300 - accuracy: 0.5952#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015245/312 [======================>.......] - ETA: 1s - loss: 1.1298 - accuracy: 0.5953#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015247/312 [======================>.......] - ETA: 1s - loss: 1.1296 - accuracy: 0.5954#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015249/312 [======================>.......] - ETA: 1s - loss: 1.1294 - accuracy: 0.5954#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015251/312 [=======================>......] - ETA: 1s - loss: 1.1292 - accuracy: 0.5955#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015253/312 [=======================>......] - ETA: 1s - loss: 1.1290 - accuracy: 0.5955#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015255/312 [=======================>......] - ETA: 1s - loss: 1.1289 - accuracy: 0.5956#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015257/312 [=======================>......] - ETA: 1s - loss: 1.1287 - accuracy: 0.5956#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015259/312 [=======================>......] - ETA: 1s - loss: 1.1285 - accuracy: 0.5957#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015261/312 [========================>.....] - ETA: 1s - loss: 1.1283 - accuracy: 0.5957#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015263/312 [========================>.....] - ETA: 1s - loss: 1.1281 - accuracy: 0.5958#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015265/312 [========================>.....] - ETA: 1s - loss: 1.1280 - accuracy: 0.5958#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015267/312 [========================>.....] - ETA: 1s - loss: 1.1278 - accuracy: 0.5959#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015269/312 [========================>.....] - ETA: 1s - loss: 1.1276 - accuracy: 0.5959#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015271/312 [=========================>....] - ETA: 1s - loss: 1.1274 - accuracy: 0.5960#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015273/312 [=========================>....] - ETA: 1s - loss: 1.1272 - accuracy: 0.5961#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015275/312 [=========================>....] - ETA: 1s - loss: 1.1270 - accuracy: 0.5961#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015277/312 [=========================>....] - ETA: 1s - loss: 1.1269 - accuracy: 0.5962#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015279/312 [=========================>....] - ETA: 0s - loss: 1.1267 - accuracy: 0.5962#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015281/312 [==========================>...] - ETA: 0s - loss: 1.1265 - accuracy: 0.5963#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015283/312 [==========================>...] - ETA: 0s - loss: 1.1263 - accuracy: 0.5963#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015285/312 [==========================>...] - ETA: 0s - loss: 1.1261 - accuracy: 0.5964#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015287/312 [==========================>...] - ETA: 0s - loss: 1.1260 - accuracy: 0.5964#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015289/312 [==========================>...] - ETA: 0s - loss: 1.1258 - accuracy: 0.5965#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015291/312 [==========================>...] - ETA: 0s - loss: 1.1256 - accuracy: 0.5966#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015293/312 [===========================>..] - ETA: 0s - loss: 1.1254 - accuracy: 0.5966#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015295/312 [===========================>..] - ETA: 0s - loss: 1.1252 - accuracy: 0.5967#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015297/312 [===========================>..] - ETA: 0s - loss: 1.1250 - accuracy: 0.5967#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015299/312 [===========================>..] - ETA: 0s - loss: 1.1248 - accuracy: 0.5968#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015301/312 [===========================>..] - ETA: 0s - loss: 1.1246 - accuracy: 0.5969#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015303/312 [============================>.] - ETA: 0s - loss: 1.1245 - accuracy: 0.5969#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015305/312 [============================>.] - ETA: 0s - loss: 1.1243 - accuracy: 0.5970#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015307/312 [============================>.] - ETA: 0s - loss: 1.1241 - accuracy: 0.5970#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015309/312 [============================>.] - ETA: 0s - loss: 1.1239 - accuracy: 0.5971#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015311/312 [============================>.] - ETA: 0s - loss: 1.1237 - accuracy: 0.5972#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015312/312 [==============================] - 10s 32ms/step - loss: 1.1236 - accuracy: 0.5972 - val_loss: 1.1507 - val_accuracy: 0.5925[0m
[34mEpoch 5/5[0m
[34m#015 1/312 [..............................] - ETA: 8s - loss: 1.1089 - accuracy: 0.5703#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 3/312 [..............................] - ETA: 9s - loss: 1.0939 - accuracy: 0.5924#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 5/312 [..............................] - ETA: 8s - loss: 1.0874 - accuracy: 0.5989#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 7/312 [..............................] - ETA: 8s - loss: 1.0759 - accuracy: 0.6038#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 9/312 [..............................] - ETA: 8s - loss: 1.0646 - accuracy: 0.6089#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 11/312 [>.............................] - ETA: 8s - loss: 1.0546 - accuracy: 0.6136#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 13/312 [>.............................] - ETA: 8s - loss: 1.0504 - accuracy: 0.6157#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 15/312 [>.............................] - ETA: 8s - loss: 1.0502 - accuracy: 0.6167#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 17/312 [>.............................] - ETA: 8s - loss: 1.0492 - accuracy: 0.6178#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 19/312 [>.............................] - ETA: 8s - loss: 1.0478 - accuracy: 0.6189#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 21/312 [=>............................] - ETA: 8s - loss: 1.0466 - accuracy: 0.6197#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 23/312 [=>............................] - ETA: 8s - loss: 1.0456 - accuracy: 0.6204#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 25/312 [=>............................] - ETA: 8s - loss: 1.0443 - accuracy: 0.6212#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 27/312 [=>............................] - ETA: 8s - loss: 1.0431 - accuracy: 0.6219#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 29/312 [=>............................] - ETA: 8s - loss: 1.0419 - accuracy: 0.6226#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 31/312 [=>............................] - ETA: 8s - loss: 1.0412 - accuracy: 0.6232#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 33/312 [==>...........................] - ETA: 8s - loss: 1.0409 - accuracy: 0.6237#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 35/312 [==>...........................] - ETA: 8s - loss: 1.0405 - accuracy: 0.6241#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 37/312 [==>...........................] - ETA: 8s - loss: 1.0402 - accuracy: 0.6245#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 39/312 [==>...........................] - ETA: 7s - loss: 1.0398 - accuracy: 0.6248#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 41/312 [==>...........................] - ETA: 7s - loss: 1.0393 - accuracy: 0.6251#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 43/312 [===>..........................] - ETA: 7s - loss: 1.0390 - accuracy: 0.6253#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 45/312 [===>..........................] - ETA: 7s - loss: 1.0387 - accuracy: 0.6255#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 47/312 [===>..........................] - ETA: 7s - loss: 1.0384 - accuracy: 0.6256#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 49/312 [===>..........................] - ETA: 7s - loss: 1.0384 - accuracy: 0.6256#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 51/312 [===>..........................] - ETA: 7s - loss: 1.0385 - accuracy: 0.6256#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 53/312 [====>.........................] - ETA: 7s - loss: 1.0386 - accuracy: 0.6257#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 55/312 [====>.........................] - ETA: 7s - loss: 1.0386 - accuracy: 0.6257#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 57/312 [====>.........................] - ETA: 7s - loss: 1.0387 - accuracy: 0.6258#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 59/312 [====>.........................] - ETA: 7s - loss: 1.0388 - accuracy: 0.6258#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 61/312 [====>.........................] - ETA: 7s - loss: 1.0389 - accuracy: 0.6258#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 63/312 [=====>........................] - ETA: 7s - loss: 1.0389 - accuracy: 0.6258#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 65/312 [=====>........................] - ETA: 7s - loss: 1.0389 - accuracy: 0.6258#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 67/312 [=====>........................] - ETA: 7s - loss: 1.0389 - accuracy: 0.6259#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 69/312 [=====>........................] - ETA: 7s - loss: 1.0388 - accuracy: 0.6260#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 71/312 [=====>........................] - ETA: 7s - loss: 1.0387 - accuracy: 0.6260#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 73/312 [======>.......................] - ETA: 6s - loss: 1.0385 - accuracy: 0.6261#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 75/312 [======>.......................] - ETA: 6s - loss: 1.0384 - accuracy: 0.6262#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 77/312 [======>.......................] - ETA: 6s - loss: 1.0383 - accuracy: 0.6263#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 79/312 [======>.......................] - ETA: 6s - loss: 1.0381 - accuracy: 0.6264#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 81/312 [======>.......................] - ETA: 6s - loss: 1.0380 - accuracy: 0.6264#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 83/312 [======>.......................] - ETA: 6s - loss: 1.0379 - accuracy: 0.6265#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 85/312 [=======>......................] - ETA: 6s - loss: 1.0377 - accuracy: 0.6266#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 87/312 [=======>......................] - ETA: 6s - loss: 1.0376 - accuracy: 0.6266#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 89/312 [=======>......................] - ETA: 6s - loss: 1.0375 - accuracy: 0.6266#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 91/312 [=======>......................] - ETA: 6s - loss: 1.0374 - accuracy: 0.6267#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 93/312 [=======>......................] - ETA: 6s - loss: 1.0373 - accuracy: 0.6267#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 95/312 [========>.....................] - ETA: 6s - loss: 1.0371 - accuracy: 0.6268#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 97/312 [========>.....................] - ETA: 6s - loss: 1.0370 - accuracy: 0.6268#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015 99/312 [========>.....................] - ETA: 6s - loss: 1.0369 - accuracy: 0.6268#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015101/312 [========>.....................] - ETA: 6s - loss: 1.0368 - accuracy: 0.6268#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015103/312 [========>.....................] - ETA: 6s - loss: 1.0367 - accuracy: 0.6269#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015105/312 [=========>....................] - ETA: 6s - loss: 1.0367 - accuracy: 0.6269#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015107/312 [=========>....................] - ETA: 5s - loss: 1.0366 - accuracy: 0.6269#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015109/312 [=========>....................] - ETA: 5s - loss: 1.0365 - accuracy: 0.6269#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015111/312 [=========>....................] - ETA: 5s - loss: 1.0364 - accuracy: 0.6270#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015113/312 [=========>....................] - ETA: 5s - loss: 1.0363 - accuracy: 0.6270#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015115/312 [==========>...................] - ETA: 5s - loss: 1.0361 - accuracy: 0.6271#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015117/312 [==========>...................] - ETA: 5s - loss: 1.0359 - accuracy: 0.6271#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015119/312 [==========>...................] - ETA: 5s - loss: 1.0358 - accuracy: 0.6271#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015121/312 [==========>...................] - ETA: 5s - loss: 1.0355 - accuracy: 0.6272#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015123/312 [==========>...................] - ETA: 5s - loss: 1.0354 - accuracy: 0.6273#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015125/312 [===========>..................] - ETA: 5s - loss: 1.0352 - accuracy: 0.6273#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015127/312 [===========>..................] - ETA: 5s - loss: 1.0350 - accuracy: 0.6274#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015129/312 [===========>..................] - ETA: 5s - loss: 1.0348 - accuracy: 0.6275#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015131/312 [===========>..................] - ETA: 5s - loss: 1.0346 - accuracy: 0.6276#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015133/312 [===========>..................] - ETA: 5s - loss: 1.0344 - accuracy: 0.6277#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015135/312 [===========>..................] - ETA: 5s - loss: 1.0342 - accuracy: 0.6277#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015137/312 [============>.................] - ETA: 5s - loss: 1.0340 - accuracy: 0.6278#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015139/312 [============>.................] - ETA: 5s - loss: 1.0338 - accuracy: 0.6279#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015141/312 [============>.................] - ETA: 4s - loss: 1.0336 - accuracy: 0.6279#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015143/312 [============>.................] - ETA: 4s - loss: 1.0334 - accuracy: 0.6280#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015145/312 [============>.................] - ETA: 4s - loss: 1.0333 - accuracy: 0.6281#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015147/312 [=============>................] - ETA: 4s - loss: 1.0331 - accuracy: 0.6281#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015149/312 [=============>................] - ETA: 4s - loss: 1.0329 - accuracy: 0.6282#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015151/312 [=============>................] - ETA: 4s - loss: 1.0327 - accuracy: 0.6282#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015153/312 [=============>................] - ETA: 4s - loss: 1.0325 - accuracy: 0.6283#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015155/312 [=============>................] - ETA: 4s - loss: 1.0323 - accuracy: 0.6284#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015157/312 [==============>...............] - ETA: 4s - loss: 1.0321 - accuracy: 0.6284#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015159/312 [==============>...............] - ETA: 4s - loss: 1.0319 - accuracy: 0.6285#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015161/312 [==============>...............] - ETA: 4s - loss: 1.0317 - accuracy: 0.6285#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015163/312 [==============>...............] - ETA: 4s - loss: 1.0315 - accuracy: 0.6286#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015165/312 [==============>...............] - ETA: 4s - loss: 1.0313 - accuracy: 0.6286#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015167/312 [===============>..............] - ETA: 4s - loss: 1.0311 - accuracy: 0.6287#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015169/312 [===============>..............] - ETA: 4s - loss: 1.0309 - accuracy: 0.6287#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015171/312 [===============>..............] - ETA: 4s - loss: 1.0308 - accuracy: 0.6288#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015173/312 [===============>..............] - ETA: 4s - loss: 1.0306 - accuracy: 0.6288#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015175/312 [===============>..............] - ETA: 3s - loss: 1.0305 - accuracy: 0.6289#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015177/312 [================>.............] - ETA: 3s - loss: 1.0303 - accuracy: 0.6289#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015179/312 [================>.............] - ETA: 3s - loss: 1.0302 - accuracy: 0.6289#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015181/312 [================>.............] - ETA: 3s - loss: 1.0300 - accuracy: 0.6290#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015183/312 [================>.............] - ETA: 3s - loss: 1.0299 - accuracy: 0.6290#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015185/312 [================>.............] - ETA: 3s - loss: 1.0297 - accuracy: 0.6291#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015187/312 [================>.............] - ETA: 3s - loss: 1.0296 - accuracy: 0.6291#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015189/312 [=================>............] - ETA: 3s - loss: 1.0294 - accuracy: 0.6292#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015191/312 [=================>............] - ETA: 3s - loss: 1.0292 - accuracy: 0.6292#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015193/312 [=================>............] - ETA: 3s - loss: 1.0291 - accuracy: 0.6293#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010[0m
[34m#010#010#010#010#010#010#010#010#010#015195/312 [=================>............] - ETA: 3s - loss: 1.0289 - accuracy: 0.6293#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015197/312 [=================>............] - ETA: 3s - loss: 1.0288 - accuracy: 0.6294#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015199/312 [==================>...........] - ETA: 3s - loss: 1.0286 - accuracy: 0.6294#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015201/312 [==================>...........] - ETA: 3s - loss: 1.0285 - accuracy: 0.6295#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015203/312 [==================>...........] - ETA: 3s - loss: 1.0283 - accuracy: 0.6296#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015205/312 [==================>...........] - ETA: 3s - loss: 1.0281 - accuracy: 0.6296#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015207/312 [==================>...........] - ETA: 3s - loss: 1.0280 - accuracy: 0.6297#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015209/312 [===================>..........] - ETA: 2s - loss: 1.0279 - accuracy: 0.6297#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015211/312 [===================>..........] - ETA: 2s - loss: 1.0277 - accuracy: 0.6298#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015213/312 [===================>..........] - ETA: 2s - loss: 1.0276 - accuracy: 0.6298#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015215/312 [===================>..........] - ETA: 2s - loss: 1.0275 - accuracy: 0.6299#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015217/312 [===================>..........] - ETA: 2s - loss: 1.0273 - accuracy: 0.6299#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015219/312 [====================>.........] - ETA: 2s - loss: 1.0272 - accuracy: 0.6300#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015221/312 [====================>.........] - ETA: 2s - loss: 1.0271 - accuracy: 0.6300#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015223/312 [====================>.........] - ETA: 2s - loss: 1.0270 - accuracy: 0.6301#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015225/312 [====================>.........] - ETA: 2s - loss: 1.0268 - accuracy: 0.6302#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015227/312 [====================>.........] - ETA: 2s - loss: 1.0267 - accuracy: 0.6302#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015229/312 [=====================>........] - ETA: 2s - loss: 1.0266 - accuracy: 0.6303#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015231/312 [=====================>........] - ETA: 2s - loss: 1.0264 - accuracy: 0.6303#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015233/312 [=====================>........] - ETA: 2s - loss: 1.0263 - accuracy: 0.6304#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015235/312 [=====================>........] - ETA: 2s - loss: 1.0262 - accuracy: 0.6304#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015237/312 [=====================>........] - ETA: 2s - loss: 1.0260 - accuracy: 0.6305#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015239/312 [=====================>........] - ETA: 2s - loss: 1.0259 - accuracy: 0.6306#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015241/312 [======================>.......] - ETA: 2s - loss: 1.0257 - accuracy: 0.6306#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015243/312 [======================>.......] - ETA: 2s - loss: 1.0256 - accuracy: 0.6307#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015245/312 [======================>.......] - ETA: 1s - loss: 1.0254 - accuracy: 0.6307#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015247/312 [======================>.......] - ETA: 1s - loss: 1.0253 - accuracy: 0.6308#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015249/312 [======================>.......] - ETA: 1s - loss: 1.0251 - accuracy: 0.6309#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015251/312 [=======================>......] - ETA: 1s - loss: 1.0250 - accuracy: 0.6309#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015253/312 [=======================>......] - ETA: 1s - loss: 1.0248 - accuracy: 0.6310#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015255/312 [=======================>......] - ETA: 1s - loss: 1.0247 - accuracy: 0.6311#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015257/312 [=======================>......] - ETA: 1s - loss: 1.0245 - accuracy: 0.6311#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015259/312 [=======================>......] - ETA: 1s - loss: 1.0243 - accuracy: 0.6312#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015261/312 [========================>.....] - ETA: 1s - loss: 1.0242 - accuracy: 0.6313#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015263/312 [========================>.....] - ETA: 1s - loss: 1.0240 - accuracy: 0.6313#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015265/312 [========================>.....] - ETA: 1s - loss: 1.0239 - accuracy: 0.6314#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015267/312 [========================>.....] - ETA: 1s - loss: 1.0237 - accuracy: 0.6314#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015269/312 [========================>.....] - ETA: 1s - loss: 1.0236 - accuracy: 0.6315#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015271/312 [=========================>....] - ETA: 1s - loss: 1.0235 - accuracy: 0.6316#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015273/312 [=========================>....] - ETA: 1s - loss: 1.0233 - accuracy: 0.6316#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015275/312 [=========================>....] - ETA: 1s - loss: 1.0232 - accuracy: 0.6317#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015277/312 [=========================>....] - ETA: 1s - loss: 1.0230 - accuracy: 0.6317#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015279/312 [=========================>....] - ETA: 0s - loss: 1.0229 - accuracy: 0.6318#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015281/312 [==========================>...] - ETA: 0s - loss: 1.0227 - accuracy: 0.6319#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015283/312 [==========================>...] - ETA: 0s - loss: 1.0226 - accuracy: 0.6319#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015285/312 [==========================>...] - ETA: 0s - loss: 1.0224 - accuracy: 0.6320#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015287/312 [==========================>...] - ETA: 0s - loss: 1.0223 - accuracy: 0.6321#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015289/312 [==========================>...] - ETA: 0s - loss: 1.0221 - accuracy: 0.6321#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015291/312 [==========================>...] - ETA: 0s - loss: 1.0220 - accuracy: 0.6322#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015293/312 [===========================>..] - ETA: 0s - loss: 1.0219 - accuracy: 0.6323#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015295/312 [===========================>..] - ETA: 0s - loss: 1.0217 - accuracy: 0.6323#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015297/312 [===========================>..] - ETA: 0s - loss: 1.0216 - accuracy: 0.6324#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015299/312 [===========================>..] - ETA: 0s - loss: 1.0214 - accuracy: 0.6325#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015301/312 [===========================>..] - ETA: 0s - loss: 1.0213 - accuracy: 0.6325#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015303/312 [============================>.] - ETA: 0s - loss: 1.0211 - accuracy: 0.6326#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015305/312 [============================>.] - ETA: 0s - loss: 1.0210 - accuracy: 0.6326#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015307/312 [============================>.] - ETA: 0s - loss: 1.0208 - accuracy: 0.6327#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015309/312 [============================>.] - ETA: 0s - loss: 1.0207 - accuracy: 0.6328#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015311/312 [============================>.] - ETA: 0s - loss: 1.0205 - accuracy: 0.6328#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#010#015312/312 [==============================] - 10s 31ms/step - loss: 1.0204 - accuracy: 0.6329 - val_loss: 0.9505 - val_accuracy: 0.6659[0m
2021-10-11 10:18:41 Uploading - Uploading generated training model
2021-10-11 10:18:41 Completed - Training job completed
[34m2021-10-11 10:17:06.915955: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m2021-10-11 10:17:06.916101: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:105] SageMaker Profiler is not enabled. The timeline writer thread will not be started, future recorded events will be dropped.[0m
[34m2021-10-11 10:17:06.957001: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34mWARNING:tensorflow:Callback method `on_test_batch_end` is slow compared to the batch time (batch time: 0.0027s vs `on_test_batch_end` time: 0.0055s). Check your callbacks.[0m
[34mWARNING:tensorflow:Callback method `on_test_batch_end` is slow compared to the batch time (batch time: 0.0027s vs `on_test_batch_end` time: 0.0055s). Check your callbacks.[0m
[34m2021-10-11 10:18:22.170449: W tensorflow/python/util/util.cc:348] Sets are not currently considered sequences, but this may change in the future, so consider avoiding using them.[0m
[34mINFO:tensorflow:Assets written to: /opt/ml/model/1/assets[0m
[34mINFO:tensorflow:Assets written to: /opt/ml/model/1/assets
[0m
[34m2021-10-11 10:18:27,253 sagemaker-training-toolkit INFO Reporting training SUCCESS[0m
Training seconds: 293
Billable seconds: 293
| MIT | code/phase0/1.2.Train_Keras_Local_Script_Mode.ipynb | gonsoomoon-ml/SageMaker-Tensorflow-Step-By-Step |
6. 모델 아티펙트 저장- S3 에 저장된 모델 아티펙트를 저장하여 추론시 사용합니다. | keras_script_artifact_path = estimator.model_data
print("script_artifact_path: ", keras_script_artifact_path)
%store keras_script_artifact_path | script_artifact_path: s3://sagemaker-us-east-1-227612457811/cifar10-2021-10-11-10-10-05-339/output/model.tar.gz
Stored 'keras_script_artifact_path' (str)
| MIT | code/phase0/1.2.Train_Keras_Local_Script_Mode.ipynb | gonsoomoon-ml/SageMaker-Tensorflow-Step-By-Step |
Add model: translation attention ecoder-decocer over the b4 dataset | import numpy as np
import torch
import torch.nn as nn
import torch.nn.functional as F
from torchtext import data
import pandas as pd
import unicodedata
import string
import re
import random
import copy
from contra_qa.plots.functions import simple_step_plot, plot_confusion_matrix
import matplotlib.pyplot as plt
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
from nltk.translate.bleu_score import sentence_bleu
% matplotlib inline
import time
import math
def asMinutes(s):
m = math.floor(s / 60)
s -= m * 60
return '%dm %ds' % (m, s)
def timeSince(since):
now = time.time()
s = now - since
return '%s' % asMinutes(s) | _____no_output_____ | MIT | lab/notebooks_phase2/AddModel_simpleB_b5.ipynb | felipessalvatore/ContraQA |
Preparing data | df2 = pd.read_csv("data/boolean5_train.csv")
df2_test = pd.read_csv("data/boolean5_test.csv")
df2["text"] = df2["sentence1"] + df2["sentence2"]
df2_test["text"] = df2_test["sentence1"] + df2_test["sentence2"]
all_sentences = list(df2.text.values) + list(df2_test.text.values)
df2train = df2.iloc[:8500]
df2valid = df2.iloc[8500:]
df2train.tail()
SOS_token = 0
EOS_token = 1
class Lang:
def __init__(self, name):
self.name = name
self.word2index = {}
self.word2count = {}
self.index2word = {0: "SOS", 1: "EOS"}
self.n_words = 2 # Count SOS and EOS
def addSentence(self, sentence):
for word in sentence.split(' '):
self.addWord(word)
def addWord(self, word):
if word not in self.word2index:
self.word2index[word] = self.n_words
self.word2count[word] = 1
self.index2word[self.n_words] = word
self.n_words += 1
else:
self.word2count[word] += 1
# Turn a Unicode string to plain ASCII, thanks to
# http://stackoverflow.com/a/518232/2809427
def unicodeToAscii(s):
return ''.join(
c for c in unicodedata.normalize('NFD', s)
if unicodedata.category(c) != 'Mn')
# Lowercase, trim, and remove non-letter characters
def normalizeString(s):
s = unicodeToAscii(s.lower().strip())
s = re.sub(r"([.!?])", r" \1", s)
s = re.sub(r"[^a-zA-Z.!?]+", r" ", s)
return s
example = "ddddda'''~~çãpoeéééééÈ'''#$$##@!@!@AAS@#12323fdf"
print("Before:", example)
print()
print("After:", normalizeString(example))
pairs_A = list(zip(list(df2train.sentence1.values), list(df2train.and_A.values)))
pairs_B = list(zip(list(df2train.sentence1.values), list(df2train.and_B.values)))
pairs_A = [(normalizeString(s1), normalizeString(s2)) for s1, s2 in pairs_A]
pairs_B = [(normalizeString(s1), normalizeString(s2)) for s1, s2 in pairs_B]
pairs_A_val = list(zip(list(df2valid.sentence1.values), list(df2valid.and_A.values)))
pairs_B_val = list(zip(list(df2valid.sentence1.values), list(df2valid.and_B.values)))
pairs_A_val = [(normalizeString(s1), normalizeString(s2)) for s1, s2 in pairs_A_val]
pairs_B_val = [(normalizeString(s1), normalizeString(s2)) for s1, s2 in pairs_B_val]
all_text_pairs = zip(all_sentences, all_sentences)
all_text_pairs = [(normalizeString(s1), normalizeString(s2)) for s1, s2 in all_text_pairs]
def readLangs(lang1, lang2, pairs, reverse=False):
# Reverse pairs, make Lang instances
if reverse:
pairs = [tuple(reversed(p)) for p in pairs]
input_lang = Lang(lang2)
output_lang = Lang(lang1)
else:
input_lang = Lang(lang1)
output_lang = Lang(lang2)
return input_lang, output_lang, pairs
f = lambda x: len(x.split(" "))
MAX_LENGTH = np.max(list(map(f, all_sentences)))
MAX_LENGTH = 20
def filterPair(p):
cond1 = len(p[0].split(' ')) < MAX_LENGTH
cond2 = len(p[1].split(' ')) < MAX_LENGTH
return cond1 and cond2
def filterPairs(pairs):
return [pair for pair in pairs if filterPair(pair)]
def prepareData(lang1, lang2, pairs, reverse=False):
input_lang, output_lang, pairs = readLangs(lang1, lang2, pairs, reverse)
print("Read %s sentence pairs" % len(pairs))
pairs = filterPairs(pairs)
print("Trimmed to %s sentence pairs" % len(pairs))
print("Counting words...")
for pair in pairs:
input_lang.addSentence(pair[0])
output_lang.addSentence(pair[1])
print("Counted words:")
print(input_lang.name, input_lang.n_words)
print(output_lang.name, output_lang.n_words)
return input_lang, output_lang, pairs
_, _, training_pairs_A = prepareData("eng_enc",
"eng_dec",
pairs_A)
print()
input_lang, _, _ = prepareData("eng_enc",
"eng_dec",
all_text_pairs)
output_lang = copy.deepcopy(input_lang)
print()
print()
_, _, valid_pairs_A = prepareData("eng_enc",
"eng_dec",
pairs_A_val)
_, _, training_pairs_B = prepareData("eng_enc",
"eng_dec",
pairs_B)
print()
_, _, valid_pairs_B = prepareData("eng_enc",
"eng_dec",
pairs_B_val) | Read 8500 sentence pairs
Trimmed to 8500 sentence pairs
Counting words...
Counted words:
eng_enc 773
eng_dec 772
Read 1500 sentence pairs
Trimmed to 1500 sentence pairs
Counting words...
Counted words:
eng_enc 701
eng_dec 700
| MIT | lab/notebooks_phase2/AddModel_simpleB_b5.ipynb | felipessalvatore/ContraQA |
sentences 2 tensors | def indexesFromSentence(lang, sentence):
return [lang.word2index[word] for word in sentence.split(' ')]
def tensorFromSentence(lang, sentence):
indexes = indexesFromSentence(lang, sentence)
indexes.append(EOS_token)
return torch.tensor(indexes, dtype=torch.long, device=device).view(-1, 1)
def tensorsFromPair(pair):
input_tensor = tensorFromSentence(input_lang, pair[0])
target_tensor = tensorFromSentence(output_lang, pair[1])
return (input_tensor, target_tensor)
def tensorsFromTriple(triple):
input_tensor = tensorFromSentence(input_lang, triple[0])
target_tensor = tensorFromSentence(output_lang, triple[1])
label_tensor = torch.tensor(triple[2], dtype=torch.long).view((1))
return (input_tensor, target_tensor, label_tensor) | _____no_output_____ | MIT | lab/notebooks_phase2/AddModel_simpleB_b5.ipynb | felipessalvatore/ContraQA |
models | class EncoderRNN(nn.Module):
def __init__(self, input_size, hidden_size):
super(EncoderRNN, self).__init__()
self.hidden_size = hidden_size
self.embedding = nn.Embedding(input_size, hidden_size)
self.gru = nn.GRU(hidden_size, hidden_size)
def forward(self, input, hidden):
embedded = self.embedding(input).view(1, 1, -1)
output = embedded
output, hidden = self.gru(output, hidden)
return output, hidden
def initHidden(self):
return torch.zeros(1, 1, self.hidden_size, device=device)
class AttnDecoderRNN(nn.Module):
def __init__(self, hidden_size, output_size, dropout_p=0.1, max_length=MAX_LENGTH):
super(AttnDecoderRNN, self).__init__()
self.hidden_size = hidden_size
self.output_size = output_size
self.dropout_p = dropout_p
self.max_length = max_length
self.embedding = nn.Embedding(self.output_size, self.hidden_size)
self.attn = nn.Linear(self.hidden_size * 2, self.max_length)
self.attn_combine = nn.Linear(self.hidden_size * 2, self.hidden_size)
self.dropout = nn.Dropout(self.dropout_p)
self.gru = nn.GRU(self.hidden_size, self.hidden_size)
self.out = nn.Linear(self.hidden_size, self.output_size)
def forward(self, input, hidden, encoder_outputs):
embedded = self.embedding(input).view(1, 1, -1)
embedded = self.dropout(embedded)
attn_weights = F.softmax(
self.attn(torch.cat((embedded[0], hidden[0]), 1)), dim=1)
attn_applied = torch.bmm(attn_weights.unsqueeze(0),
encoder_outputs.unsqueeze(0))
output = torch.cat((embedded[0], attn_applied[0]), 1)
output = self.attn_combine(output).unsqueeze(0)
output = F.relu(output)
output, hidden = self.gru(output, hidden)
output = F.log_softmax(self.out(output[0]), dim=1)
return output, hidden, attn_weights
def initHidden(self):
return torch.zeros(1, 1, self.hidden_size, device=device)
hidden_size = 256
eng_enc_v_size = input_lang.n_words
eng_dec_v_size = output_lang.n_words
input_lang.n_words
encoderA = EncoderRNN(eng_enc_v_size, hidden_size)
decoderA = AttnDecoderRNN(hidden_size, eng_dec_v_size)
encoderA.load_state_dict(torch.load("b5_encoder1_att.pkl"))
decoderA.load_state_dict(torch.load("b5_decoder1_att.pkl"))
encoderB = EncoderRNN(eng_enc_v_size, hidden_size)
decoderB = AttnDecoderRNN(hidden_size, eng_dec_v_size)
encoderB.load_state_dict(torch.load("b5_encoder2_att.pkl"))
decoderB.load_state_dict(torch.load("b5_decoder2_att.pkl")) | _____no_output_____ | MIT | lab/notebooks_phase2/AddModel_simpleB_b5.ipynb | felipessalvatore/ContraQA |
translating | def translate(encoder,
decoder,
sentence,
max_length=MAX_LENGTH):
with torch.no_grad():
input_tensor = tensorFromSentence(input_lang, sentence)
input_length = input_tensor.size()[0]
encoder_hidden = encoder.initHidden()
encoder_outputs = torch.zeros(
max_length, encoder.hidden_size, device=device)
for ei in range(input_length):
encoder_output, encoder_hidden = encoder(input_tensor[ei],
encoder_hidden)
encoder_outputs[ei] += encoder_output[0, 0]
decoder_input = torch.tensor([[SOS_token]], device=device) # SOS
decoder_hidden = encoder_hidden
decoded_words = []
for di in range(max_length):
decoder_output, decoder_hidden, decoder_attention = decoder(decoder_input, decoder_hidden, encoder_outputs)
_, topone = decoder_output.data.topk(1)
if topone.item() == EOS_token:
decoded_words.append('<EOS>')
break
else:
decoded_words.append(output_lang.index2word[topone.item()])
decoder_input = topone.squeeze().detach()
return " ".join(decoded_words) | _____no_output_____ | MIT | lab/notebooks_phase2/AddModel_simpleB_b5.ipynb | felipessalvatore/ContraQA |
translation of a trained model: and A | for t in training_pairs_A[0:3]:
print("input_sentence : " + t[0])
neural_translation = translate(encoderA,
decoderA,
t[0],
max_length=MAX_LENGTH)
print("neural translation : " + neural_translation)
reference = t[1] + ' <EOS>'
print("reference translation : " + reference)
reference = reference.split(" ")
candidate = neural_translation.split(" ")
score = sentence_bleu([reference], candidate)
print("blue score = {:.2f}".format(score))
print() | input_sentence : jeffery created a silly and vast work of art
neural translation : brenda created a blue work of art <EOS>
reference translation : jeffery created a silly work of art <EOS>
blue score = 0.41
input_sentence : hilda created a zealous and better work of art
neural translation : brenda created a pitiful work of art <EOS>
reference translation : hilda created a zealous work of art <EOS>
blue score = 0.41
input_sentence : cheryl created an ugly and obedient work of art
neural translation : brenda created an ugly work of art <EOS>
reference translation : cheryl created an ugly work of art <EOS>
blue score = 0.84
| MIT | lab/notebooks_phase2/AddModel_simpleB_b5.ipynb | felipessalvatore/ContraQA |
translation of a trained model: and B | for t in training_pairs_B[0:3]:
print("input_sentence : " + t[0])
neural_translation = translate(encoderB,
decoderB,
t[0],
max_length=MAX_LENGTH)
print("neural translation : " + neural_translation)
reference = t[1] + ' <EOS>'
print("reference translation : " + reference)
reference = reference.split(" ")
candidate = neural_translation.split(" ")
score = sentence_bleu([reference], candidate)
print("blue score = {:.2f}".format(score))
print() | input_sentence : jeffery created a silly and vast work of art
neural translation : marion created a vast work of art <EOS>
reference translation : jeffery created a vast work of art <EOS>
blue score = 0.84
input_sentence : hilda created a zealous and better work of art
neural translation : marion created a better work of art <EOS>
reference translation : hilda created a better work of art <EOS>
blue score = 0.84
input_sentence : cheryl created an ugly and obedient work of art
neural translation : jessie created an obedient work of art <EOS>
reference translation : cheryl created an obedient work of art <EOS>
blue score = 0.84
| MIT | lab/notebooks_phase2/AddModel_simpleB_b5.ipynb | felipessalvatore/ContraQA |
Defining the And modelmodel inner working:- $s_1$ is the first sentence (e.g., 'penny is thankful and naomi is alive')- $s_2$ is the second sentence (e.g., 'penny is not alive')- $h_A = dec_{A}(enc_{A}(s_1, \vec{0}))$- $h_B = dec_{B}(enc_{B}(s_1, \vec{0}))$- $h_{inf} = \sigma (W[h_A ;h_B] + b)$- $e = enc_{A}(s_2, h_{inf})$- $\hat{y} = softmax(We + b)$ | class AndModel(nn.Module):
def __init__(self,
encoderA,
decoderA,
encoderB,
decoderB,
hidden_size,
output_size,
max_length,
input_lang,
target_lang,
SOS_token=0,
EOS_token=1):
super(AndModel, self).__init__()
self.max_length = max_length
self.hidden_size = hidden_size
self.output_size = output_size
self.encoderA = encoderA
self.decoderA = decoderA
self.encoderB = encoderB
self.decoderB = decoderB
self.input_lang = input_lang
self.target_lang = target_lang
self.SOS_token = SOS_token
self.EOS_token = EOS_token
self.fc_inf = nn.Linear(hidden_size * 2, hidden_size)
self.fc_out = nn.Linear(hidden_size, output_size)
def encode(self,
sentence,
encoder,
is_tensor,
hidden=None):
if not is_tensor:
input_tensor = tensorFromSentence(self.input_lang, sentence)
else:
input_tensor = sentence
input_length = input_tensor.size()[0]
if hidden is None:
encoder_hidden = encoder.initHidden()
else:
encoder_hidden = hidden
encoder_outputs = torch.zeros(self.max_length,
encoder.hidden_size,
device=device)
for ei in range(input_length):
encoder_output, encoder_hidden = encoder(input_tensor[ei],
encoder_hidden)
encoder_outputs[ei] += encoder_output[0, 0]
self.encoder_outputs = encoder_outputs
return encoder_hidden
def decode(self,
tensor,
decoder,
out_tensor):
decoder_input = torch.tensor([[self.SOS_token]], device=device)
decoder_hidden = tensor
decoded_words = []
for di in range(self.max_length):
decoder_output, decoder_hidden, decoder_attention = decoder(
decoder_input, decoder_hidden, self.encoder_outputs)
_, topone = decoder_output.data.topk(1)
if topone.item() == self.EOS_token:
decoded_words.append('<EOS>')
break
else:
decoded_words.append(self.target_lang.index2word[topone.item()])
decoder_input = topone.squeeze().detach()
if not out_tensor:
output = " ".join(decoded_words)
else:
output = decoder_hidden
return output
def sen2vec(self, sentence, encoder, decoder, is_tensor, out_tensor):
encoded = self.encode(sentence, encoder, is_tensor)
vec = self.decode(encoded, decoder, out_tensor)
return vec
def sen2vecA(self, sentence, is_tensor):
encoded = self.encode(sentence, self.encoderA, is_tensor)
vec = self.decode(encoded, self.decoderA, out_tensor=True)
return vec
def sen2vecB(self, sentence, is_tensor):
encoded = self.encode(sentence, self.encoderB, is_tensor)
vec = self.decode(encoded, self.decoderB, out_tensor=True)
return vec
def forward(self, s1, s2):
hA = self.sen2vecA(s1, is_tensor=True)
hB = self.sen2vecB(s1, is_tensor=True)
# h_inf = torch.cat([hA, hB], dim=2).squeeze(1)
# h_inf = torch.sigmoid(self.fc_inf(h_inf))
# h_inf = h_inf.view((1, h_inf.shape[0], h_inf.shape[1]))
h_inf = hA
e = self.encode(s2,
self.encoderA,
hidden=h_inf,
is_tensor=True)
output = self.fc_out(e).squeeze(1)
return output
def predict(self, s1, s2):
out = self.forward(s1, s2)
softmax = nn.Softmax(dim=1)
out = softmax(out)
indices = torch.argmax(out, 1)
return indices
addmodel = AndModel(encoderA,
decoderA,
encoderB,
decoderB,
hidden_size=256,
output_size=2,
max_length=MAX_LENGTH,
input_lang=input_lang,
target_lang=output_lang) | _____no_output_____ | MIT | lab/notebooks_phase2/AddModel_simpleB_b5.ipynb | felipessalvatore/ContraQA |
Test encoding decoding | for ex in training_pairs_B[0:3]:
print("===========")
ex = ex[0]
print("s1:\n")
print(ex)
print()
ex_A = addmodel.sen2vec(ex,
addmodel.encoderA,
addmodel.decoderA,
is_tensor=False,
out_tensor=False)
ex_B = addmodel.sen2vec(ex,
addmodel.encoderB,
addmodel.decoderB,
is_tensor=False,
out_tensor=False)
print("inference A:\n")
print(ex_A)
print()
print("inference B:\n")
print(ex_B)
for ex in training_pairs_B[0:1]:
print("===========")
ex = ex[0]
print("s1:\n")
print(ex)
print()
ex_A = addmodel.sen2vecA(ex,is_tensor=False)
ex_B = addmodel.sen2vecB(ex,is_tensor=False)
print(ex_A)
print()
print(ex_B)
train_triples = zip(list(df2train.sentence1.values), list(df2train.sentence2.values), list(df2train.label.values))
train_triples = [(normalizeString(s1), normalizeString(s2), l) for s1, s2, l in train_triples]
train_triples_t = [tensorsFromTriple(t) for t in train_triples]
train_triples = zip(list(df2train.sentence1.values), list(df2train.sentence2.values), list(df2train.label.values))
train_triples = [(normalizeString(s1), normalizeString(s2), l) for s1, s2, l in train_triples]
train_triples_t = [tensorsFromTriple(t) for t in train_triples]
valid_triples = zip(list(df2valid.sentence1.values), list(df2valid.sentence2.values), list(df2valid.label.values))
valid_triples = [(normalizeString(s1), normalizeString(s2), l) for s1, s2, l in valid_triples]
valid_triples_t = [tensorsFromTriple(t) for t in valid_triples]
len(valid_triples_t)
test_triples = zip(list(df2_test.sentence1.values), list(df2_test.sentence2.values), list(df2_test.label.values))
test_triples = [(normalizeString(s1), normalizeString(s2), l) for s1, s2, l in test_triples]
test_triples_t = [tensorsFromTriple(t) for t in test_triples]
example = train_triples[0]
print(example)
example_t = train_triples_t[0]
print(example_t) | ('jeffery created a silly and vast work of art', 'jeffery didn t create a silly work of art', 1)
(tensor([[ 2],
[ 3],
[ 4],
[ 5],
[ 6],
[ 7],
[ 8],
[ 9],
[10],
[ 1]]), tensor([[ 2],
[11],
[12],
[13],
[ 4],
[ 5],
[ 8],
[ 9],
[10],
[ 1]]), tensor([1]))
| MIT | lab/notebooks_phase2/AddModel_simpleB_b5.ipynb | felipessalvatore/ContraQA |
Prediction BEFORE training | n_iters = 100
training_pairs_little = [random.choice(train_triples_t) for i in range(n_iters)]
predictions = []
labels = []
for i in range(n_iters):
s1, s2, label = training_pairs_little[i]
pred = addmodel.predict(s1, s2)
label = label.item()
pred = pred.item()
predictions.append(pred)
labels.append(label)
plot_confusion_matrix(labels,
predictions,
classes=["no", "yes"],
path="confusion_matrix.png") | _____no_output_____ | MIT | lab/notebooks_phase2/AddModel_simpleB_b5.ipynb | felipessalvatore/ContraQA |
Training functions | def CEtrain(s1_tensor,
s2_tensor,
label,
model,
optimizer,
criterion):
model.train()
optimizer.zero_grad()
logits = model(s1_tensor, s2_tensor)
loss = criterion(logits, label)
loss.backward()
optimizer.step()
return loss | _____no_output_____ | MIT | lab/notebooks_phase2/AddModel_simpleB_b5.ipynb | felipessalvatore/ContraQA |
Test CEtrain | CE = nn.CrossEntropyLoss()
addmodel_opt = torch.optim.SGD(addmodel.parameters(), lr= 0.3)
loss = CEtrain(s1_tensor=example_t[0],
s2_tensor=example_t[1],
label=example_t[2],
model=addmodel,
optimizer=addmodel_opt,
criterion=CE)
assert type(loss.item()) == float | _____no_output_____ | MIT | lab/notebooks_phase2/AddModel_simpleB_b5.ipynb | felipessalvatore/ContraQA |
Little example of training | epochs = 10
learning_rate = 0.1
CE = nn.CrossEntropyLoss()
encoderA = EncoderRNN(eng_enc_v_size, hidden_size)
decoderA = AttnDecoderRNN(hidden_size, eng_dec_v_size)
encoderA.load_state_dict(torch.load("b5_encoder1_att.pkl"))
decoderA.load_state_dict(torch.load("b5_decoder1_att.pkl"))
encoderB = EncoderRNN(eng_enc_v_size, hidden_size)
decoderB = AttnDecoderRNN(hidden_size, eng_dec_v_size)
encoderB.load_state_dict(torch.load("b5_encoder2_att.pkl"))
decoderB.load_state_dict(torch.load("b5_decoder2_att.pkl"))
addmodel = AndModel(encoderA,
decoderA,
encoderB,
decoderB,
hidden_size=256,
output_size=2,
max_length=MAX_LENGTH,
input_lang=input_lang,
target_lang=output_lang)
# # for model in [encoderA, decoderA, encoderB, decoderB]:
# for model in [encoderB, decoderB]:
# for param in model.parameters():
# param.requires_grad = False
# addmodel_opt = torch.optim.SGD(addmodel.parameters(), lr= learning_rate)
addmodel_opt = torch.optim.Adagrad(addmodel.parameters(), lr= learning_rate)
# addmodel_opt = torch.optim.Adadelta(addmodel.parameters(), lr= learning_rate)
# addmodel_opt = torch.optim.Adam(addmodel.parameters(), lr= learning_rate)
# addmodel_opt = torch.optim.SparseAdam(addmodel.parameters(), lr= learning_rate)
# addmodel_opt = torch.optim.RMSprop(addmodel.parameters(), lr= learning_rate)
losses_per_epoch = []
for i in range(epochs):
losses = []
start = time.time()
n_iters = 1000
training_pairs_little = [random.choice(train_triples_t) for i in range(n_iters)]
for t in training_pairs_little:
s1, s2, label = t
loss = CEtrain(s1_tensor=s1,
s2_tensor=s2,
label=label,
model=addmodel,
optimizer=addmodel_opt,
criterion=CE)
losses.append(loss.item())
mean_loss = np.mean(losses)
losses_per_epoch.append(mean_loss)
print("epoch {}/{}".format(i+1, epochs), timeSince(start), "mean loss = {:.2f}".format(mean_loss))
simple_step_plot([losses_per_epoch],
"loss",
"loss example ({} epochs)".format(epochs),
"loss_example.png",
figsize=(15,4)) | epoch 1/10 0m 59s mean loss = 1.36
epoch 2/10 0m 58s mean loss = 0.87
epoch 3/10 1m 2s mean loss = 0.85
epoch 4/10 0m 59s mean loss = 0.82
epoch 5/10 0m 54s mean loss = 0.80
epoch 6/10 0m 53s mean loss = 0.78
epoch 7/10 0m 50s mean loss = 0.77
epoch 8/10 0m 59s mean loss = 0.77
epoch 9/10 0m 53s mean loss = 0.76
epoch 10/10 0m 52s mean loss = 0.77
| MIT | lab/notebooks_phase2/AddModel_simpleB_b5.ipynb | felipessalvatore/ContraQA |
Prediction AFTER training | n_iters = 100
training_pairs_little = [random.choice(train_triples_t) for i in range(n_iters)]
predictions = []
labels = []
for i in range(n_iters):
s1, s2, label = training_pairs_little[i]
pred = addmodel.predict(s1, s2)
label = label.item()
pred = pred.item()
predictions.append(pred)
labels.append(label)
plot_confusion_matrix(labels,
predictions,
classes=["no", "yes"],
path="confusion_matrix.png")
n_iters = len(valid_triples_t)
valid_pairs_little = [random.choice(valid_triples_t) for i in range(n_iters)]
predictions = []
labels = []
for i in range(n_iters):
s1, s2, label = valid_pairs_little[i]
pred = addmodel.predict(s1, s2)
label = label.item()
pred = pred.item()
predictions.append(pred)
labels.append(label)
plot_confusion_matrix(labels,
predictions,
classes=["no", "yes"],
path="confusion_matrix.png")
torch.save(addmodel.state_dict(), "b5_simpleB.pkl")
n_iters = len(test_triples_t)
test_pairs_little = [random.choice(test_triples_t) for i in range(n_iters)]
predictions = []
labels = []
for i in range(n_iters):
s1, s2, label = test_pairs_little[i]
pred = addmodel.predict(s1, s2)
label = label.item()
pred = pred.item()
predictions.append(pred)
labels.append(label)
plot_confusion_matrix(labels,
predictions,
classes=["no", "yes"],
path="confusion_matrix.png") | _____no_output_____ | MIT | lab/notebooks_phase2/AddModel_simpleB_b5.ipynb | felipessalvatore/ContraQA |
 Ingeniería de características Suavizado de series de tiempo [Julio Waissman Vilanova]([email protected])Vamos a ver diferentes tipos y formas de suavisar curvas. Para esto, vamos a utilizar como serie de tiempo la serie de casos confirmados por COVID-19 recabados y mantenidos por [Luis Armando Moreno](http://www.luisarmandomoreno.com), los cuales se pueden descargar [aquí](https://onedrive.live.com/download.aspx?resid=5ADDF6870413EAC9!40221&authkey=!AHWUE_EQfhvGRm4). | import pandas as pd
import statsmodels.api as sm
import plotly.graph_objects as go
import plotly.express as px
confirmados = pd.read_csv(
"Casosdiarios.csv",
engine="python",
parse_dates=['Fecha']
)[['Fecha', 'CASOS']] \
.groupby("Fecha") \
.sum() \
.diff() + 1
fig = px.scatter(
confirmados,
y="CASOS"
).update_layout(
title='Casos confirmados de COVID-10 en Sonora',
hovermode="x"
).show() | _____no_output_____ | MIT | suavizado/suavizado.ipynb | mcd-unison/ing-caracteristicas-2020 |
Suavizado por medias movilesEl suavizado por media movil utiliza una ventana de tiempo en los datos para suavizar. La ventana de tiempo debe de tener sentido para los datos, pero se puede jugar con ella. Para esto se usa el método `rolling` el cual se puede usar con otros tipos de funciones. | confirmados["ma 3"] = confirmados.CASOS.rolling(window=3, center=True).mean()
confirmados["ma 7"] = confirmados.CASOS.rolling(window=7, center=True).mean()
confirmados["ma 14"] = confirmados.CASOS.rolling(window=14, center=True).mean()
fig = go.Figure(
).add_scatter(
x=confirmados.index,
y=confirmados["CASOS"],
mode='markers',
name="Real"
).add_scatter(
x=confirmados.index,
y=confirmados["ma 3"],
hovertemplate="%{y:.1f}",
name="MA 3 días"
).add_scatter(
x=confirmados.index,
y=confirmados["ma 7"],
hovertemplate="%{y:.1f}",
name="MA 7 días"
).add_scatter(
x=confirmados.index,
y=confirmados["ma 14"],
hovertemplate="%{y:.1f}",
name="MA 14 días"
).update_layout(
title='Casos confirmados de COVID-10 en Sonora',
hovermode="x unified"
).show() | _____no_output_____ | MIT | suavizado/suavizado.ipynb | mcd-unison/ing-caracteristicas-2020 |
Medianas moviles exponenciales | confirmados["mm 3"] = confirmados.CASOS.rolling(window=3, center=True).median()
confirmados["mm 7"] = confirmados.CASOS.rolling(window=7, center=True).median()
confirmados["mm 14"] = confirmados.CASOS.rolling(window=14, center=True).median()
fig = go.Figure(
).add_scatter(
x=confirmados.index,
y=confirmados["CASOS"],
mode='markers',
name="Real"
).add_scatter(
x=confirmados.index,
y=confirmados["mm 3"],
hovertemplate="%{y:.1f}",
name="MM 3 días"
).add_scatter(
x=confirmados.index,
y=confirmados["mm 7"],
hovertemplate="%{y:.1f}",
name="MM 7 días"
).add_scatter(
x=confirmados.index,
y=confirmados["mm 14"],
hovertemplate="%{y:.1f}",
name="MM 14 días"
).update_layout(
title='Casos confirmados de COVID-10 en Sonora',
hovermode="x"
).show() | _____no_output_____ | MIT | suavizado/suavizado.ipynb | mcd-unison/ing-caracteristicas-2020 |
Medias moviles exponenciales | confirmados["ewm 3"] = confirmados.CASOS.ewm(span=3).mean()
confirmados["ewm 7"] = confirmados.CASOS.ewm(span=7).mean()
confirmados["ewm 14"] = confirmados.CASOS.ewm(span=14).mean()
fig = go.Figure(
).add_scatter(
x=confirmados.index,
y=confirmados["CASOS"],
mode='markers',
name="Real"
).add_scatter(
x=confirmados.index,
y=confirmados["ewm 3"],
hovertemplate="%{y:.1f}",
name="EWM 3 días"
).add_scatter(
x=confirmados.index,
y=confirmados["ewm 7"],
hovertemplate="%{y:.1f}",
name="EWM 7 días"
).add_scatter(
x=confirmados.index,
y=confirmados["ewm 14"],
hovertemplate="%{y:.1f}",
name="EWM 14 días"
).update_layout(
title='Casos confirmados de COVID-10 en Sonora'
).show() | _____no_output_____ | MIT | suavizado/suavizado.ipynb | mcd-unison/ing-caracteristicas-2020 |
LOWESS | lowess = sm.nonparametric.lowess
l1 = lowess(confirmados.CASOS, confirmados.index, frac=1/5)
l2 = lowess(confirmados.CASOS, confirmados.index, frac=1/10)
l3 = lowess(confirmados.CASOS, confirmados.index, frac=1/20)
fig = go.Figure(
).add_scatter(
x=confirmados.index,
y=confirmados["CASOS"],
mode='markers',
name="Real"
).add_scatter(
x=confirmados.index[1:],
y=l1[:,1],
hovertemplate="%{y:.1f}",
name="LOWESS 1/5"
).add_scatter(
x=confirmados.index[1:],
y=l2[:,1],
hovertemplate="%{y:.1f}",
name="LOWESS 1/10"
).add_scatter(
x=confirmados.index[1:],
y=l3[:,1],
hovertemplate="%{y:.1f}",
name="LOWESS 1/20"
).update_layout(
title='Casos confirmados de COVID-10 en Sonora',
hovermode="x"
).show()
fig = go.Figure(
).add_scatter(
x=confirmados.index,
y=confirmados["CASOS"],
mode='markers',
name="Real"
).add_scatter(
x=confirmados.index[1:],
y=l2[:,0],
hovertemplate="%{y:.1f}",
name="LOWESS 1/5"
).add_scatter(
x=confirmados.index[1:],
y=l2[:,1],
hovertemplate="%{y:.1f}",
name="LOWESS 1/10"
).update_layout(
title='Casos confirmados de COVID-10 en Sonora',
hovermode="x"
).show()
fig = go.Figure(
).add_scatter(
x=confirmados.index,
y=confirmados["CASOS"],
mode='markers',
name="Real"
).add_scatter(
x=confirmados.index,
y=confirmados["ma 7"],
hovertemplate="%{y:.1f}",
name="MA 7 días"
).add_scatter(
x=confirmados.index,
y=confirmados["mm 7"],
hovertemplate="%{y:.1f}",
name="MM 7 días"
).add_scatter(
x=confirmados.index,
y=confirmados["ewm 7"],
hovertemplate="%{y:.1f}",
name="EWM 7 días"
).add_scatter(
x=confirmados.index[1:],
y=l2[:,1],
hovertemplate="%{y:.1f}",
name="LOWESS 1/10"
).update_layout(
title='Casos confirmados de COVID-10 en Sonora',
hovermode="x"
).show()
| _____no_output_____ | MIT | suavizado/suavizado.ipynb | mcd-unison/ing-caracteristicas-2020 |
Casting as a date type | %%read_sql
SELECT date::date
FROM wnv_train
LIMIT 10 | Query started at 02:52:58 PM EDT; Query executed in 0.00 m | MIT | src/solutions/feature_engineering_solutions.ipynb | crawles/data-science-training |
Extracting year, month, and day from a date | input_table = 'wnv_train'
output_table = 'wnv_train_ts'
%%read_sql
DROP TABLE if EXISTS {output_table};
CREATE TABLE {output_table}
AS
WITH wnv_train_dates AS (
SELECT date::date date_ts, *
FROM {input_table}
)
SELECT extract(year from date_ts)::int AS year,
extract(month from date_ts)::int AS month,
extract(day from date_ts)::int AS day,
extract(doy from date_ts)::int AS day_of_year,
*
FROM wnv_train_dates
%read_sql SELECT * FROM {output_table} LIMIT 10 | Query started at 02:52:59 PM EDT; Query executed in 0.00 m | MIT | src/solutions/feature_engineering_solutions.ipynb | crawles/data-science-training |
Aggregate to Trap, Species, Level We are asked to predict for a given day, trap, and species, predict the presence of West Nile Virus in mosquitoes. | input_table = 'wnv_train_ts'
output_table = 'wnv_train_agg'
%%read_sql
DROP TABLE IF EXISTS {output_table};
CREATE TABLE {output_table}
AS
SELECT date_ts,year,month,day,day_of_year,trap,latitude,longitude,species,
SUM(NumMosquitos)::int total_num_mosquitos,
MAX(WnvPresent) wnv_present
FROM {input_table}
GROUP BY date_ts,year,month,day,day_of_year,trap,latitude,longitude,species
%%read_sql
SELECT *
FROM {output_table} | Query started at 02:53:00 PM EDT; Query executed in 0.00 m | MIT | src/solutions/feature_engineering_solutions.ipynb | crawles/data-science-training |
Categorical Variable Enconding | input_table = 'wnv_train_agg'
output_table = 'wnv_train_agg_dummy'
df = %read_sql SELECT species FROM {input_table}
import re
species = ['CULEX PIPIENS/RESTUANS',
'CULEX RESTUANS',
'CULEX PIPIENS',
'CULEX TERRITANS',
'CULEX SALINARIUS',
'CULEX TARSALIS',
'CULEX ERRATICUS']
def _clean_dummy_val( cval):
"""For creating dummy variable columns, we need to remove special characters in values"""
cval_clean = cval.replace(' ','_').replace('-','_').replace('"','').replace("'",'')
return re.sub('[^a-zA-Z\d\s:]', '_', cval_clean)
def to_dummy(c, c_value):
c_val_clean = _clean_dummy_val(c_value)
return "CAST({c}='{c_value}' AS int) AS {c}_{c_val_clean}".format(c=c,
c_value = c_value,
c_val_clean = c_val_clean
)
cols = %read_sql SELECT * FROM {input_table} LIMIT 0
cols = cols.columns.tolist()
cols.remove('species')
sql = cols + [to_dummy('species', c) for c in species]
sql_str = ',\n'.join(sql)
%%read_sql
DROP TABLE IF EXISTS {output_table};
CREATE TABLE {output_table}
AS
SELECT {sql_str}
FROM {input_table};
%read_sql SELECT madlib.create_indicator_variables()
%%read_sql
DROP TABLE IF EXISTS {output_table};
SELECT madlib.create_indicator_variables('{input_table}',
'{output_table}',
'species')
%read_sql SELECT * FROM {output_table} | Query started at 02:54:09 PM EDT; Query executed in 0.00 m | MIT | src/solutions/feature_engineering_solutions.ipynb | crawles/data-science-training |
Weather Data Data Cleansing and Missing ValuesNeed to remove whitespace and replace characters with empty | input_table = 'wnv_weather'
output_table = 'wnv_trimmed'
df = %read_sql SELECT * FROM wnv_weather
df.dtypes | Query started at 03:08:37 PM EDT; Query executed in 0.00 m | MIT | src/solutions/feature_engineering_solutions.ipynb | crawles/data-science-training |
Trim White Space | %%read_sql
DROP TABLE IF EXISTS {output_table};
CREATE TEMP TABLE {output_table}
AS
SELECT *,
trim(avgspeed) avgspeed_trimmed,
trim(preciptotal) preciptotal_trimmed,
/* trim(tmin) tmin_trimmed, */
trim(tavg) tavg_trimmed
/* trim(tmax) tmax_trimmed */
FROM {input_table}
%read_sql SELECT * FROM wnv_trimmed
conn.commit() | _____no_output_____ | MIT | src/solutions/feature_engineering_solutions.ipynb | crawles/data-science-training |
Replacing Missing Variables | input_table = 'wnv_trimmed'
output_table = 'wnv_weather_clean'
%%read_sql
DROP TABLE IF EXISTS {output_table};
CREATE TABLE {output_table}
AS
SELECT date,
CAST(regexp_replace(avgspeed_trimmed, '^[^\d.]+$', '0') AS float) AS avgspeed,
CAST(regexp_replace(preciptotal_trimmed, '^[^\d.]+$', '0') AS float) AS preciptotal,
CAST(regexp_replace(tavg_trimmed, '^[^\\d.]+$'::text, '0') AS float) AS tavg,
tmin,
tmax
FROM {input_table} | Query started at 03:15:26 PM EDT; Query executed in 0.00 mQuery started at 03:15:26 PM EDT; Query executed in 0.00 m | MIT | src/solutions/feature_engineering_solutions.ipynb | crawles/data-science-training |
Create weather date features (same as for station data)This analysis is the same as used for the station data so we have filled it in. | input_table = 'wnv_weather_clean'
output_table = 'wnv_weather_ts'
%%read_sql
DROP TABLE if EXISTS {output_table};
CREATE TABLE {output_table}
AS
WITH wnv_weather_dates AS (
SELECT date::date date_ts, *
FROM {input_table}
)
SELECT extract(year from date_ts)::int AS year,
extract(month from date_ts)::int AS month,
extract(day from date_ts)::int AS day,
extract(doy from date_ts)::int AS day_of_year,
*
FROM wnv_weather_dates
%%read_sql
SELECT *
FROM {output_table}
LIMIT 10 | Query started at 03:15:30 PM EDT; Query executed in 0.00 m | MIT | src/solutions/feature_engineering_solutions.ipynb | crawles/data-science-training |
Compute Weather Averages | input_table = 'wnv_weather_ts'
output_table = 'wnv_weather_rolling'
%%read_sql
DROP TABLE IF EXISTS {output_table};
CREATE TABLE {output_table}
AS
SELECT date_ts,
avg(avgspeed) OVER (ORDER BY date_ts RANGE BETWEEN 7 PRECEDING AND CURRENT ROW),
avg(preciptotal) OVER (ORDER BY date_ts RANGE BETWEEN 7 PRECEDING AND CURRENT ROW) AS preciptotal7,
avg(tmin) OVER (ORDER BY date_ts RANGE BETWEEN 7 PRECEDING AND CURRENT ROW) AS tmin7,
avg(tavg) OVER (ORDER BY date_ts RANGE BETWEEN 7 PRECEDING AND CURRENT ROW) AS tavg7,
avg(tmax) OVER (ORDER BY date_ts RANGE BETWEEN 7 PRECEDING AND CURRENT ROW) AS tmax7
FROM {input_table}
ORDER BY date_ts
%%read_sql
SELECT *
FROM {output_table}
LIMIT 10 | Query started at 03:52:39 PM Eastern Daylight Time
Query executed in 0.00 m
| MIT | src/solutions/feature_engineering_solutions.ipynb | crawles/data-science-training |
Putting it All Together: Join weather data and mosquito station data | station_table = 'wnv_train_agg_dummy'
weather_table = 'wnv_weather_rolling'
output_table = 'wnv_features'
df = %read_sql SELECT * FROM {station_table} LIMIT 10
df.columns.tolist()
%%read_sql
DROP TABLE IF EXISTS {output_table};
CREATE TABLE {output_table}
AS
SELECT row_number() OVER () as id, *
FROM {station_table}
INNER JOIN {weather_table}
USING (date_ts);
print(1) | 1
| MIT | src/solutions/feature_engineering_solutions.ipynb | crawles/data-science-training |
(FCD)= 1.5 Definición de función, continuidad y derivada ```{admonition} Notas para contenedor de docker:Comando de docker para ejecución de la nota de forma local:nota: cambiar `` por la ruta de directorio que se desea mapear a `/datos` dentro del contenedor de docker y `` por la versión más actualizada que se presenta en la documentación.`docker run --rm -v :/datos --name jupyterlab_optimizacion -p 8888:8888 -d palmoreck/jupyterlab_optimizacion:`password para jupyterlab: `qwerty`Detener el contenedor de docker:`docker stop jupyterlab_optimizacion`Documentación de la imagen de docker `palmoreck/jupyterlab_optimizacion:` en [liga](https://github.com/palmoreck/dockerfiles/tree/master/jupyterlab/optimizacion).``` --- Nota generada a partir de la [liga1](https://www.dropbox.com/s/jfrxanjls8kndjp/Diferenciacion_e_Integracion.pdf?dl=0), [liga2](https://www.dropbox.com/s/mmd1uzvwhdwsyiu/4.3.2.Teoria_de_convexidad_Funciones_convexas.pdf?dl=0) e inicio de [liga3](https://www.dropbox.com/s/ko86cce1olbtsbk/4.3.1.Teoria_de_convexidad_Conjuntos_convexos.pdf?dl=0). ```{admonition} Al final de esta nota el y la lectora::class: tip* Aprenderá las definiciones de función y derivada de una función en algunos casos de interés para el curso. En específico el caso de derivada direccional es muy importante.* Aprenderá que el gradiente y Hessiana de una función son un vector y una matriz de primeras (información de primer orden) y segundas derivadas (información de segundo orden) respectivamente.* Aprenderá algunas fórmulas utilizadas con el operador nabla de diferenciación.* Aprenderá la diferencia entre el cálculo algebraico o simbólico y el numérico vía el paquete *SymPy*.``` Función ```{admonition} DefiniciónUna función, $f$, es una regla de correspondencia entre un conjunto nombrado dominio y otro conjunto nombrado codominio.``` Notación$f: A \rightarrow B$ es una función de un conjunto $\text{dom}f \subseteq A$ en un conjunto $B$.```{admonition} Observación:class: tip$\text{dom}f$ (el dominio de $f$) puede ser un subconjunto propio de $A$, esto es, algunos elementos de $A$ y otros no, son mapeados a elementos de $B$.``` En lo que sigue se considera al espacio $\mathbb{R}^n$ y se asume que conjuntos y subconjuntos están en este espacio. (CACCI)= Conjunto abierto, cerrado, cerradura e interior ```{margin} Un punto $x$ se nombra **punto límite** de un conjunto $X$, si existe una sucesión $\{x_k\} \subset X$ que converge a $x$. El conjunto de puntos límites se nombra **cerradura** o *closure* de $X$ y se denota como $\text{cl}X$. Un conjunto $X$ se nombra **cerrado** si es igual a su cerradura.``` ```{admonition} DefiniciónEl interior de un conjunto $X$ es el conjunto de **puntos interiores**: un punto $x$ de un conjunto $X$ se llama interior si existe una **vecindad** de $x$ (conjunto abierto\* que contiene a $x$) contenida en $X$.\*Un conjunto $X$ se dice que es **abierto** si $\forall x \in X$ existe una bola abierta\* centrada en $x$ y contenida en $X$. Es equivalente escribir que $X$ es **abierto** si su complemento $\mathbb{R}^n \ X$ es cerrado.\*Una **bola abierta** con radio $\epsilon>0$ y centrada en $x$ es el conjunto: $B_\epsilon(x) =\{y \in \mathbb{R}^n : ||y-x|| ` para ejemplos de bolas abiertas en el plano.``` En lo siguiente $\text{intdom}f$ es el **interior** del dominio de $f$. Continuidad ```{admonition} Definición$f: \mathbb{R}^n \rightarrow \mathbb{R}^m$ es continua en $x \in \text{dom}f$ si $\forall \epsilon >0 \exists \delta > 0$ tal que:$$y \in \text{dom}f, ||y-x||_2 \leq \delta \implies ||f(y)-f(x)||_2 \leq \epsilon$$``` ```{admonition} Comentarios* $f$ continua en un punto $x$ del dominio de $f$ entonces $f(y)$ es arbitrariamente cercana a $f(x)$ para $y$ en el dominio de $f$ cercana a $x$.* Otra forma de definir que $f$ sea continua en $x \in \text{dom}f$ es con sucesiones y límites: si $\{x_i\}_{i \in \mathbb{N}} \subseteq \text{dom}f$ es una sucesión de puntos en el dominio de $f$ que converge a $x \in \text{dom}f$, $\displaystyle \lim_{i \rightarrow \infty}x_i = x$, y $f$ es continua en $x$ entonces la sucesión $\{f(x_i)\}_{i \in \mathbb{N}}$ converge a $f(x)$: $\displaystyle \lim_{i \rightarrow \infty}f(x_i) = f(x) = f \left(\displaystyle \lim_{i \rightarrow \infty} x_i \right )$.``` Notación$\mathcal{C}([a,b])=\{\text{funciones } f:\mathbb{R} \rightarrow \mathbb{R} \text{ continuas en el intervalo [a,b]}\}$ y $\mathcal{C}(\text{dom}f) = \{\text{funciones } f:\mathbb{R}^n \rightarrow \mathbb{R}^m \text{ continuas en su dominio}\}$. Función Diferenciable Caso $f: \mathbb{R} \rightarrow \mathbb{R}$ ```{admonition} Definición$f$ es diferenciable en $x_0 \in (a,b)$ si $\displaystyle \lim_{x \rightarrow x_0} \frac{f(x)-f(x_0)}{x-x_0}$ existe y escribimos:$$f^{(1)}(x_0) = \displaystyle \lim_{x \rightarrow x_0} \frac{f(x)-f(x_0)}{x-x_0}.$$``` $f$ es diferenciable en $[a,b]$ si es diferenciable en cada punto de $[a,b]$. Análogamente definiendo la variable $h=x-x_0$ se tiene: $f^{(1)}(x_0) = \displaystyle \lim_{h \rightarrow 0} \frac{f(x_0+h)-f(x_0)}{h}$ que típicamente se escribe como:$$f^{(1)}(x) = \displaystyle \lim_{h \rightarrow 0} \frac{f(x+h)-f(x)}{h}.$$ ```{admonition} ComentarioSi $f$ es diferenciable en $x_0$ entonces $f(x) \approx f(x_0) + f^{(1)}(x_0)(x-x_0)$. Gráficamente:``` Como las derivadas también son funciones tenemos una notación para las derivadas que son continuas: Notación$\mathcal{C}^n([a,b])=\{\text{funciones } f:\mathbb{R} \rightarrow \mathbb{R} \text{ con } n \text{ derivadas continuas en el intervalo [a,b]}\}$. En Python podemos utilizar el paquete [SymPy](https://www.sympy.org/en/index.html) para calcular límites y derivadas de forma **simbólica** (ver [sympy/calculus](https://docs.sympy.org/latest/tutorial/calculus.html)) que es diferente al cálculo **numérico** que se revisa en {ref}`Polinomios de Taylor y diferenciación numérica `. Ejemplo | import sympy | _____no_output_____ | Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
**Límite de $\frac{\cos(x+h) - \cos(x)}{h}$ para $h \rightarrow 0$:** | x, h = sympy.symbols("x, h")
quotient = (sympy.cos(x+h) - sympy.cos(x))/h
sympy.pprint(sympy.limit(quotient, h, 0)) | -sin(x)
| Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
Lo anterior corresponde a la **derivada de $\cos(x)$**: | x = sympy.Symbol('x')
sympy.pprint(sympy.cos(x).diff(x)) | -sin(x)
| Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
**Si queremos evaluar la derivada podemos usar:** | sympy.pprint(sympy.cos(x).diff(x).subs(x,sympy.pi/2))
sympy.pprint(sympy.Derivative(sympy.cos(x), x))
sympy.pprint(sympy.Derivative(sympy.cos(x), x).doit_numerically(sympy.pi/2)) | -1.00000000000000
| Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
Caso $f: \mathbb{R}^n \rightarrow \mathbb{R}^m$ ```{admonition} Definición$f$ es diferenciable en $x \in \text{intdom}f$ si existe una matriz $Df(x) \in \mathbb{R}^{m\times n}$ tal que:$$\displaystyle \lim_{z \rightarrow x, z \neq x} \frac{||f(z)-f(x)-Df(x)(z-x)||_2}{||z-x||_2} = 0, z \in \text{dom}f$$en este caso $Df(x)$ se llama la derivada de $f$ en $x$.``` ```{admonition} Observación:class: tipSólo puede existir a lo más una matriz que satisfaga el límite anterior.``` ```{margin}Una función afín es de la forma $h(x) = Ax+b$ con $A \in \mathbb{R}^{p \times n}$ y $b \in \mathbb{R}^p$. Ver [Affine_transformation](https://en.wikipedia.org/wiki/Affine_transformation)``` ```{admonition} Comentarios:* $Df(x)$ también es llamada la **Jacobiana** de $f$.* Se dice que $f$ es diferenciable si $\text{dom}f$ es abierto y es diferenciable en cada punto de $\text{dom}f.$* La función: $f(x) + Df(x)(z-x)$ es afín y se le llama **aproximación de orden $1$** de $f$ en $x$ (o también cerca de $x$). Para $z$ cercana a $x$ ésta aproximación es cercana a $f(z)$.* $Df(x)$ puede encontrarse con la definición de límite anterior o con las derivadas parciales: $Df(x)_{ij} = \frac{\partial f_i(x)}{\partial x_j}, i=1,\dots,m, j=1,\dots,n$ definidas como:$$\frac{\partial f_i(x)}{\partial x_j} = \displaystyle \lim_{h \rightarrow 0} \frac{f_i(x+he_j)-f_i(x)}{h}$$donde: $f_i : \mathbb{R}^n \rightarrow \mathbb{R}$, $i=1,\dots,m,j=1,\dots,n$ y $e_j$ $j$-ésimo vector canónico que tiene un número $1$ en la posición $j$ y $0$ en las entradas restantes.* Si $f: \mathbb{R}^n \rightarrow \mathbb{R}, Df(x) \in \mathbb{R}^{1\times n}$, su transpuesta se llama **gradiente**, se denota $\nabla f(x)$, es una función $\nabla f : \mathbb{R}^n \rightarrow \mathbb{R}^n$, recibe un vector y devuelve un vector columna y sus componentes son derivadas parciales: $$\nabla f(x) = Df(x)^T = \left[ \begin{array}{c} \frac{\partial f(x)}{\partial x_1}\\ \vdots\\ \frac{\partial f(x)}{\partial x_n} \end{array} \right] = \left[ \begin{array}{c} \displaystyle \lim_{h \rightarrow 0} \frac{f(x+he_1) - f(x)}{h}\\ \vdots\\ \displaystyle \lim_{h \rightarrow 0} \frac{f(x+he_n) - f(x)}{h} \end{array} \right] \in \mathbb{R}^{n\times 1}.$$ * En este contexto, la aproximación de primer orden a $f$ en $x$ es: $f(x) + \nabla f(x)^T(z-x)$ para $z$ cercana a $x$.``` Notación$\mathcal{C}^n(\text{dom}f) = \{\text{funciones } f:\mathbb{R}^n \rightarrow \mathbb{R}^m \text{ con } n \text{ derivadas continuas en su dominio}\}$. Ejemplo$f : \mathbb{R}^2 \rightarrow \mathbb{R}^2$ dada por:$$f(x) = \left [ \begin{array}{c}x_1x_2 + x_2^2\\x_1^2 + 2x_1x_2 + x_2^2\\\end{array}\right ]$$con $x = (x_1, x_2)^T$. Calcular la derivada de $f$. | x1, x2 = sympy.symbols("x1, x2") | _____no_output_____ | Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
**Definimos funciones $f_1, f_2$ que son componentes del vector $f(x)$**. | f1 = x1*x2 + x2**2
sympy.pprint(f1)
f2 = x1**2 + x2**2 + 2*x1*x2
sympy.pprint(f2) | 2 2
x₁ + 2⋅x₁⋅x₂ + x₂
| Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
**Derivadas parciales:** Para $f_1(x) = x_1x_2 + x_2^2$: ```{margin}**Derivada parcial de $f_1$ respecto a $x_1$.**``` | df1_x1 = f1.diff(x1)
sympy.pprint(df1_x1) | x₂
| Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
```{margin}**Derivada parcial de $f_1$ respecto a $x_2$.**``` | df1_x2 = f1.diff(x2)
sympy.pprint(df1_x2) | x₁ + 2⋅x₂
| Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
Para $f_2(x) = x_1^2 + 2x_1 x_2 + x_2^2$: ```{margin}**Derivada parcial de $f_2$ respecto a $x_1$.**``` | df2_x1 = f2.diff(x1)
sympy.pprint(df2_x1) | 2⋅x₁ + 2⋅x₂
| Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
```{margin}**Derivada parcial de $f_2$ respecto a $x_2$.**``` | df2_x2 = f2.diff(x2)
sympy.pprint(df2_x2) | 2⋅x₁ + 2⋅x₂
| Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
**Entonces la derivada es:** $$Df(x) = \left [\begin{array}{cc}x_2 & x_1+2x_2\\2x_1 + 2x_2 & 2x_1+2x_2\end{array}\right ]$$ **Otra opción más fácil es utilizando [Matrices](https://docs.sympy.org/latest/tutorial/matrices.html):** | f = sympy.Matrix([f1, f2])
sympy.pprint(f) | ⎡ 2 ⎤
⎢ x₁⋅x₂ + x₂ ⎥
⎢ ⎥
⎢ 2 2⎥
⎣x₁ + 2⋅x₁⋅x₂ + x₂ ⎦
| Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
```{margin} **Jacobiana de $f$**``` | sympy.pprint(f.jacobian([x1, x2])) | ⎡ x₂ x₁ + 2⋅x₂ ⎤
⎢ ⎥
⎣2⋅x₁ + 2⋅x₂ 2⋅x₁ + 2⋅x₂⎦
| Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
**Para evaluar por ejemplo en $(x_1, x_2)^T = (0, 1)^T$:** | d = f.jacobian([x1, x2])
sympy.pprint(d.subs([(x1, 0), (x2, 1)])) | ⎡1 2⎤
⎢ ⎥
⎣2 2⎦
| Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
Regla de la cadena ```{admonition} DefiniciónSi $f:\mathbb{R}^n \rightarrow \mathbb{R}^m$ es diferenciable en $x\in \text{intdom}f$ y $g:\mathbb{R}^m \rightarrow \mathbb{R}^p$ es diferenciable en $f(x)\in \text{intdom}g$, se define la composición $h:\mathbb{R}^n \rightarrow \mathbb{R}^p$ por $h(z) = g(f(z))$, la cual es diferenciable en $x$, con derivada:$$Dh(x)=Dg(f(x))Df(x)\in \mathbb{R}^{p\times n}.$$``` (EJ1)= EjemploSean $f:\mathbb{R}^n \rightarrow \mathbb{R}$, $g:\mathbb{R} \rightarrow \mathbb{R}$, $h:\mathbb{R}^n \rightarrow \mathbb{R}$ con $h(z) = g(f(z))$ entonces: $$Dh(x) = Dg(f(x))Df(x) = \frac{dg(f(x))}{dx}\nabla f(x)^T \in \mathbb{R}^{1\times n}$$y la transpuesta de $Dh(x)$ es: $\nabla h(x) = Dh(x)^T = \frac{dg(f(x))}{dx} \nabla f(x) \in \mathbb{R}^{n\times 1}$. Ejemplo$f(x) = \cos(x), g(x)=\sin(x)$ por lo que $h(x) = \sin(\cos(x))$. Calcular la derivada de $h$. | x = sympy.Symbol('x')
f = sympy.cos(x)
sympy.pprint(f)
g = sympy.sin(x)
sympy.pprint(g)
h = g.subs(x, f)
sympy.pprint(h)
sympy.pprint(h.diff(x)) | -sin(x)⋅cos(cos(x))
| Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
**Otras formas para calcular la derivada de la composición $h$:** | g = sympy.sin
h = g(f)
sympy.pprint(h.diff(x))
h = sympy.sin(f)
sympy.pprint(h.diff(x)) | -sin(x)⋅cos(cos(x))
| Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
Ejemplo$f(x) = x_1 + \frac{1}{x_2}, g(x) = e^x$ por lo que $h(x) = e^{x_1 + \frac{1}{x_2}}$. Calcular la derivada de $h$. | x1, x2 = sympy.symbols("x1, x2")
f = x1 + 1/x2
sympy.pprint(f)
g = sympy.exp
sympy.pprint(g)
h = g(f)
sympy.pprint(h) | 1
x₁ + ──
x₂
ℯ
| Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
```{margin}**Derivada parcial de $h$ respecto a $x_1$.**``` | sympy.pprint(h.diff(x1)) | 1
x₁ + ──
x₂
ℯ
| Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
```{margin}**Derivada parcial de $h$ respecto a $x_2$.**``` | sympy.pprint(h.diff(x2)) | 1
x₁ + ──
x₂
-ℯ
──────────
2
x₂
| Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
**Otra forma para calcular el gradiente de $h$ (derivada de $h$) es utilizando [how-to-get-the-gradient-and-hessian-sympy](https://stackoverflow.com/questions/39558515/how-to-get-the-gradient-and-hessian-sympy):** | from sympy.tensor.array import derive_by_array
sympy.pprint(derive_by_array(h, (x1, x2))) | ⎡ 1 ⎤
⎢ 1 x₁ + ── ⎥
⎢ x₁ + ── x₂ ⎥
⎢ x₂ -ℯ ⎥
⎢ℯ ──────────⎥
⎢ 2 ⎥
⎣ x₂ ⎦
| Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
(CP1)= Caso particularSean:* $f: \mathbb{R}^n \rightarrow \mathbb{R}^m$, $f(x) = Ax +b$ con $A \in \mathbb{R}^{m\times n},b \in \mathbb{R}^m$,* $g:\mathbb{R}^m \rightarrow \mathbb{R}^p$, * $h: \mathbb{R}^n \rightarrow \mathbb{R}^p$, $h(x)=g(f(x))=g(Ax+b)$ con $\text{dom}h=\{z \in \mathbb{R}^n | Az+b \in \text{dom}g\}$ entonces:$$Dh(x) = Dg(f(x))Df(x)=Dg(Ax+b)A.$$ ```{admonition} Observación:class: tipSi $p=1$, $g: \mathbb{R}^m \rightarrow \mathbb{R}$, $h: \mathbb{R}^n \rightarrow \mathbb{R}$ se tiene:$$\nabla h(x) = Dh(x)^T = A^TDg(Ax+b)^T=A^T\nabla g(Ax+b) \in \mathbb{R}^{n\times 1}.$$``` (EJRestriccionALinea)= EjemploEste caso particular considera un caso importante en el que se tienen funciones restringidas a una línea. Si $f: \mathbb{R}^n \rightarrow \mathbb{R}$, $g: \mathbb{R} \rightarrow \mathbb{R}$ está dada por $g(t) = f(x+tv)$ con $x, v \in \mathbb{R}^n$ y $t \in \mathbb{R}$, entonces escribimos que $g$ es $f$ pero restringida a la línea $x+tv$. La derivada de $g$ es:$$Dg(t) = \nabla f(x+tv)^T v.$$El escalar $Dg(0) = \nabla f(x)^Tv$ se llama **derivada direccional** de $f$ en $x$ en la dirección $v$. Un dibujo en el que se considera $\Delta x: = v$: Como ejemplo considérese $f(x) = x_1 ^2 + x_2^2$ con $x=(x_1, x_2)^T$ y $g(t) = f(x+tv)$ para $v=(v_1, v_2)^T$ vector fijo y $t \in \mathbb{R}$. Calcular $Dg(t)$. **Primera opción** | x1, x2 = sympy.symbols("x1, x2")
f = x1**2 + x2**2
sympy.pprint(f)
t = sympy.Symbol('t')
v1, v2 = sympy.symbols("v1, v2")
new_args_for_f_function = {"x1": x1+t*v1, "x2": x2 + t*v2}
g = f.subs(new_args_for_f_function)
sympy.pprint(g) | 2 2
(t⋅v₁ + x₁) + (t⋅v₂ + x₂)
| Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
```{margin} **Derivada de $g$ respecto a $t$: $Dg(t)=\nabla f(x+tv)^T v$.**``` | sympy.pprint(g.diff(t)) | 2⋅v₁⋅(t⋅v₁ + x₁) + 2⋅v₂⋅(t⋅v₂ + x₂)
| Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
**Segunda opción para calcular la derivada utilizando vectores:** | x = sympy.Matrix([x1, x2])
sympy.pprint(x)
v = sympy.Matrix([v1, v2])
new_arg_f_function = x+t*v
sympy.pprint(new_arg_f_function)
mapping_for_g_function = {"x1": new_arg_f_function[0],
"x2": new_arg_f_function[1]}
g = f.subs(mapping_for_g_function)
sympy.pprint(g) | 2 2
(t⋅v₁ + x₁) + (t⋅v₂ + x₂)
| Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
```{margin} **Derivada de $g$ respecto a $t$: $Dg(t)=\nabla f(x+tv)^T v$.**``` | sympy.pprint(g.diff(t)) | 2⋅v₁⋅(t⋅v₁ + x₁) + 2⋅v₂⋅(t⋅v₂ + x₂)
| Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
**Tercera opción definiendo a la función $f$ a partir de $x$ symbol Matrix:** | sympy.pprint(x)
f = x[0]**2 + x[1]**2
sympy.pprint(f)
sympy.pprint(new_arg_f_function)
g = f.subs({"x1": new_arg_f_function[0],
"x2": new_arg_f_function[1]})
sympy.pprint(g) | 2 2
(t⋅v₁ + x₁) + (t⋅v₂ + x₂)
| Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
```{margin} **Derivada de $g$ respecto a $t$: $Dg(t)=\nabla f(x+tv)^T v$.**``` | sympy.pprint(g.diff(t)) | 2⋅v₁⋅(t⋅v₁ + x₁) + 2⋅v₂⋅(t⋅v₂ + x₂)
| Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
**En lo siguiente se utiliza [derive-by_array](https://docs.sympy.org/latest/modules/tensor/array.htmlderivatives-by-array), [how-to-get-the-gradient-and-hessian-sympy](https://stackoverflow.com/questions/39558515/how-to-get-the-gradient-and-hessian-sympy) para mostrar cómo se puede hacer un producto punto con SymPy** | sympy.pprint(derive_by_array(f, x))
sympy.pprint(derive_by_array(f, x).subs({"x1": new_arg_f_function[0],
"x2": new_arg_f_function[1]}))
gradient_f_new_arg = derive_by_array(f, x).subs({"x1": new_arg_f_function[0],
"x2": new_arg_f_function[1]})
sympy.pprint(v) | ⎡v₁⎤
⎢ ⎥
⎣v₂⎦
| Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
```{margin} **Derivada de $g$ respecto a $t$: $Dg(t)=\nabla f(x+tv)^T v = v^T \nabla f(x + tv)$.**``` | sympy.pprint(v.dot(gradient_f_new_arg)) | v₁⋅(2⋅t⋅v₁ + 2⋅x₁) + v₂⋅(2⋅t⋅v₂ + 2⋅x₂)
| Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
(EJ2)= EjemploSi $h: \mathbb{R}^n \rightarrow \mathbb{R}$ dada por $h(x) = \log \left( \displaystyle \sum_{i=1}^m \exp(a_i^Tx+b_i) \right)$ con $x\in \mathbb{R}^n,a_i\in \mathbb{R}^n \forall i=1,\dots,m$ y $b_i \in \mathbb{R} \forall i=1,\dots,m$ entonces: $$Dh(x)=\left(\displaystyle \sum_{i=1}^m\exp(a_i^Tx+b_i) \right)^{-1}\left[ \begin{array}{c} \exp(a_1^Tx+b_1)\\ \vdots\\ \exp(a_m^Tx+b_m) \end{array} \right]^TA=(1^Tz)^{-1}z^TA$$donde: $A=(a_i)_{i=1}^m \in \mathbb{R}^{m\times n}, b \in \mathbb{R}^m$, $z=\left[ \begin{array}{c} \exp(a_1^Tx+b_1)\\ \vdots\\ \exp(a_m^Tx+b_m) \end{array}\right]$. Por lo tanto $\nabla h(x) = (1^Tz)^{-1}A^Tz$. En este ejemplo $Dh(x) = Dg(f(x))Df(x)$ con:* $h(x)=g(f(x))$,* $g: \mathbb{R}^m \rightarrow \mathbb{R}$ dada por $g(y)=\log \left( \displaystyle \sum_{i=1}^m \exp(y_i) \right )$,* $f(x)=Ax+b.$ Para lo siguiente se utilizó como referencias: [liga1](https://stackoverflow.com/questions/41581002/how-to-derive-with-respect-to-a-matrix-element-with-sympy), [liga2](https://docs.sympy.org/latest/modules/tensor/indexed.html), [liga3](https://stackoverflow.com/questions/37705571/sum-over-matrix-entries-in-sympy-1-0-with-python-3-5), [liga4](https://docs.sympy.org/latest/modules/tensor/array.html), [liga5](https://docs.sympy.org/latest/modules/concrete.html), [liga6](https://stackoverflow.com/questions/51723550/summation-over-a-sympy-array). | m = sympy.Symbol('m')
n = sympy.Symbol('n') | _____no_output_____ | Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
```{margin} Ver [indexed](https://docs.sympy.org/latest/modules/tensor/indexed.html)``` | y = sympy.IndexedBase('y')
i = sympy.Symbol('i') #for index of sum
g = sympy.log(sympy.Sum(sympy.exp(y[i]), (i, 1, m))) | _____no_output_____ | Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
```{margin} **Esta función es la que queremos derivar.**``` | sympy.pprint(g) | ⎛ m ⎞
⎜ ___ ⎟
⎜ ╲ ⎟
⎜ ╲ y[i]⎟
log⎜ ╱ ℯ ⎟
⎜ ╱ ⎟
⎜ ‾‾‾ ⎟
⎝i = 1 ⎠
| Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
**Para un caso de $m=3$ en la función $g$ se tiene:** | y1, y2, y3 = sympy.symbols("y1, y2, y3")
g_m_3 = sympy.log(sympy.exp(y1) + sympy.exp(y2) + sympy.exp(y3))
sympy.pprint(g_m_3) | ⎛ y₁ y₂ y₃⎞
log⎝ℯ + ℯ + ℯ ⎠
| Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
```{margin} Ver [derive-by_array](https://docs.sympy.org/latest/modules/tensor/array.htmlderivatives-by-array)``` | dg_m_3 = derive_by_array(g_m_3, [y1, y2, y3]) | _____no_output_____ | Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
```{margin} **Derivada de $g$ respecto a $y_1, y_2, y_3$.** ``` | sympy.pprint(dg_m_3) | ⎡ y₁ y₂ y₃ ⎤
⎢ ℯ ℯ ℯ ⎥
⎢─────────────── ─────────────── ───────────────⎥
⎢ y₁ y₂ y₃ y₁ y₂ y₃ y₁ y₂ y₃⎥
⎣ℯ + ℯ + ℯ ℯ + ℯ + ℯ ℯ + ℯ + ℯ ⎦
| Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
```{margin} Ver [Kronecker delta](https://en.wikipedia.org/wiki/Kronecker_delta)``` | sympy.pprint(derive_by_array(g, [y[1], y[2], y[3]])) | ⎡ m m m ⎤
⎢ ____ ____ ____ ⎥
⎢ ╲ ╲ ╲ ⎥
⎢ ╲ ╲ ╲ ⎥
⎢ ╲ y[i] ╲ y[i] ╲ y[i] ⎥
⎢ ╱ ℯ ⋅δ ╱ ℯ ⋅δ ╱ ℯ ⋅δ ⎥
⎢ ╱ 1,i ╱ 2,i ╱ 3,i⎥
⎢ ╱ ╱ ╱ ⎥
⎢ ‾‾‾‾ ‾‾‾‾ ‾‾‾‾ ⎥
⎢i = 1 i = 1 i = 1 ⎥
⎢──────────────── ──────────────── ────────────────⎥
⎢ m m m ⎥
⎢ ___ ___ ___ ⎥
⎢ ╲ ╲ ╲ ⎥
⎢ ╲ y[i] ╲ y[i] ╲ y[i] ⎥
⎢ ╱ ℯ ╱ ℯ ╱ ℯ ⎥
⎢ ╱ ╱ ╱ ⎥
⎢ ‾‾‾ ‾‾‾ ‾‾‾ ⎥
⎣ i = 1 i = 1 i = 1 ⎦
| Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
**Para la composición $h(x) = g(f(x))$ se utilizan las siguientes celdas:** ```{margin} Ver [indexed](https://docs.sympy.org/latest/modules/tensor/indexed.html)``` | A = sympy.IndexedBase('A')
x = sympy.IndexedBase('x')
j = sympy.Symbol('j')
b = sympy.IndexedBase('b')
#we want something like:
sympy.pprint(sympy.exp(sympy.Sum(A[i, j]*x[j], (j, 1, n)) + b[i]))
#better if we split each step:
arg_sum = A[i, j]*x[j]
sympy.pprint(arg_sum)
arg_exp = sympy.Sum(arg_sum, (j, 1, n)) + b[i]
sympy.pprint(arg_exp)
sympy.pprint(sympy.exp(arg_exp))
arg_2_sum = sympy.exp(arg_exp)
sympy.pprint(sympy.Sum(arg_2_sum, (i, 1, m)))
h = sympy.log(sympy.Sum(arg_2_sum, (i, 1, m)))
#complex expression: sympy.log(sympy.Sum(sympy.exp(sympy.Sum(A[i, j]*x[j], (j, 1, n)) + b[i]), (i, 1, m)))
sympy.pprint(h) | ⎛ m ⎞
⎜_______ ⎟
⎜╲ ⎟
⎜ ╲ ⎟
⎜ ╲ n ⎟
⎜ ╲ ___ ⎟
⎜ ╲ ╲ ⎟
⎜ ╲ ╲ ⎟
log⎜ ╱ b[i] + ╱ A[i, j]⋅x[j]⎟
⎜ ╱ ╱ ⎟
⎜ ╱ ‾‾‾ ⎟
⎜ ╱ j = 1 ⎟
⎜ ╱ ℯ ⎟
⎜╱ ⎟
⎜‾‾‾‾‾‾‾ ⎟
⎝ i = 1 ⎠
| Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
```{margin} **Derivada de $h$ respecto a $x_1$.**``` | sympy.pprint(h.diff(x[1])) | m
________
╲
╲ n
╲ ___
╲ ╲
╲ ╲
╲ b[i] + ╱ A[i, j]⋅x[j] n
╲ ╱ ___
╱ ‾‾‾ ╲
╱ j = 1 ╲ δ ⋅A[i, j]
╱ ℯ ⋅ ╱ 1,j
╱ ╱
╱ ‾‾‾
╱ j = 1
╱
‾‾‾‾‾‾‾‾
i = 1
──────────────────────────────────────────────────────
m
_______
╲
╲
╲ n
╲ ___
╲ ╲
╲ ╲
╱ b[i] + ╱ A[i, j]⋅x[j]
╱ ╱
╱ ‾‾‾
╱ j = 1
╱ ℯ
╱
‾‾‾‾‾‾‾
i = 1
| Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
```{margin} Ver [Kronecker delta](https://en.wikipedia.org/wiki/Kronecker_delta)``` | sympy.pprint(derive_by_array(h, [x[1]])) #we can use also: derive_by_array(h, [x[1], x[2], x[3]] | ⎡ m ⎤
⎢________ ⎥
⎢╲ ⎥
⎢ ╲ n ⎥
⎢ ╲ ___ ⎥
⎢ ╲ ╲ ⎥
⎢ ╲ ╲ ⎥
⎢ ╲ b[i] + ╱ A[i, j]⋅x[j] n ⎥
⎢ ╲ ╱ ___ ⎥
⎢ ╱ ‾‾‾ ╲ ⎥
⎢ ╱ j = 1 ╲ δ ⋅A[i, j]⎥
⎢ ╱ ℯ ⋅ ╱ 1,j ⎥
⎢ ╱ ╱ ⎥
⎢ ╱ ‾‾‾ ⎥
⎢ ╱ j = 1 ⎥
⎢╱ ⎥
⎢‾‾‾‾‾‾‾‾ ⎥
⎢ i = 1 ⎥
⎢──────────────────────────────────────────────────────⎥
⎢ m ⎥
⎢ _______ ⎥
⎢ ╲ ⎥
⎢ ╲ ⎥
⎢ ╲ n ⎥
⎢ ╲ ___ ⎥
⎢ ╲ ╲ ⎥
⎢ ╲ ╲ ⎥
⎢ ╱ b[i] + ╱ A[i, j]⋅x[j] ⎥
⎢ ╱ ╱ ⎥
⎢ ╱ ‾‾‾ ⎥
⎢ ╱ j = 1 ⎥
⎢ ╱ ℯ ⎥
⎢ ╱ ⎥
⎢ ‾‾‾‾‾‾‾ ⎥
⎣ i = 1 ⎦
| Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
```{admonition} Pregunta:class: tip¿Se puede resolver este ejercicio con [Matrix Symbol](https://docs.sympy.org/latest/modules/matrices/expressions.html)?``` ```{admonition} Ejercicio:class: tipVerificar que lo obtenido con SymPy es igual a lo desarrollado en "papel" al inicio del {ref}`Ejemplo ```` Segunda derivada de una función $f: \mathbb{R}^n \rightarrow \mathbb{R}$. ```{admonition} DefiniciónSea $f:\mathbb{R}^n \rightarrow \mathbb{R}$. La segunda derivada o matriz **Hessiana** de $f$ en $x \in \text{intdom}f$ existe si $f$ es dos veces diferenciable en $x$, se denota $\nabla^2f(x)$ y sus componentes son segundas derivadas parciales:$$\nabla^2f(x) = \left[\begin{array}{cccc}\frac{\partial^2f(x)}{\partial x_1^2} &\frac{\partial^2f(x)}{\partial x_2 \partial x_1}&\dots&\frac{\partial^2f(x)}{\partial x_n \partial x_1}\\\frac{\partial^2f(x)}{\partial x_1 \partial x_2} &\frac{\partial^2f(x)}{\partial x_2^2} &\dots&\frac{\partial^2f(x)}{\partial x_n \partial x_2}\\\vdots &\vdots& \ddots&\vdots\\\frac{\partial^2f(x)}{\partial x_1 \partial x_n} &\frac{\partial^2f(x)}{\partial x_2 \partial x_n}&\dots&\frac{\partial^2f(x)}{\partial x_n^2} \\\end{array}\right]$$``` ```{admonition} Comentarios:* La aproximación de segundo orden a $f$ en $x$ (o también para puntos cercanos a $x$) es la función cuadrática en la variable $z$:$$f(x) + \nabla f(x)^T(z-x)+\frac{1}{2}(z-x)^T\nabla^2f(x)(z-x)$$* Se cumple:$$\displaystyle \lim_{z \rightarrow x, z \neq x} \frac{|f(z)-[f(x)+\nabla f(x)^T(z-x)+\frac{1}{2}(z-x)^T\nabla^2f(x)(z-x)]|}{||z-x||_2} = 0, z \in \text{dom}f$$* Se tiene lo siguiente: * $\nabla f$ es una función nombrada *gradient mapping* (o simplemente gradiente). * $\nabla f:\mathbb{R}^n \rightarrow \mathbb{R}^n$ tiene regla de correspondencia $\nabla f(x)$ (evaluar en $x$ la matriz $Df(\cdot)^T$). * Se dice que $f$ es dos veces diferenciable en $\text{dom}f$ si $\text{dom}f$ es abierto y $f$ es dos veces diferenciable en cada punto de $x$. * $D\nabla f(x) = \nabla^2f(x)$ para $x \in \text{intdom}f$. * $\nabla ^2 f(x) : \mathbb{R}^n \rightarrow \mathbb{R}^{n \times n}$. * Si $f \in \mathcal{C}^2(\text{dom}f)$ entonces la Hessiana es una matriz simétrica. ``` Regla de la cadena para la segunda derivada (CP2)= Caso particular Sean:* $f:\mathbb{R}^n \rightarrow \mathbb{R}$, * $g:\mathbb{R} \rightarrow \mathbb{R}$, * $h:\mathbb{R}^n \rightarrow \mathbb{R}$ con $h(x) = g(f(x))$, entonces: $$\nabla^2h(x) = D\nabla h(x)$$ ```{margin} Ver {ref}`Ejemplo 1 de la regla de la cadena ` ``` y $$\nabla h(x)=Dh(x)^T = (Dg(f(x))Df(x))^T=\frac{dg(f(x))}{dx}\nabla f(x)$$ por lo que:$$\begin{eqnarray}\nabla^2 h(x) &=& D\nabla h(x) \nonumber \\&=& D \left(\frac{dg(f(x))}{dx}\nabla f(x)\right) \nonumber \\&=& \frac{dg(f(x))}{dx}\nabla^2 f(x)+\left(\frac{d^2g(f(x))}{dx}\nabla f(x) \nabla f(x)^T \right)^T \nonumber \\&=& \frac{dg(f(x))}{dx}\nabla^2 f(x)+\frac{d^2g(f(x))}{dx} \nabla f(x) \nabla f(x)^T \nonumber\end{eqnarray}$$ (CP3)= Caso particular Sean:* $f:\mathbb{R}^n \rightarrow \mathbb{R}^m, f(x) = Ax+b$ con $A \in \mathbb{R}^{m\times n}$, $b \in \mathbb{R}^m$,* $g:\mathbb{R}^m \rightarrow \mathbb{R}^p$,* $h:\mathbb{R}^n \rightarrow \mathbb{R}^p$, $h(x) = g(f(x)) = g(Ax+b)$ con $\text{dom}h=\{z \in \mathbb{R}^n | Az+b \in \text{dom}g\}$ entonces: ```{margin}Ver {ref}`Caso particular ` para la expresión de la derivada.``` $$Dh(x)^T = Dg(f(x))Df(x) = Dg(Ax+b)A.$$ ```{admonition} Observación:class: tipSi $p=1$, $g: \mathbb{R}^m \rightarrow \mathbb{R}$, $h: \mathbb{R}^n \rightarrow \mathbb{R}$ se tiene: $$\nabla^2h(x) = D \nabla h(x) = A^T \nabla^2g(Ax+b)A.$$``` Ejemplo ```{margin}Ver {ref}`Ejemplo ```` Si $f:\mathbb{R}^n \rightarrow \mathbb{R}$, $g: \mathbb{R} \rightarrow \mathbb{R}$ está dada por $g(t) = f(x+tv)$ con $x,v \in \mathbb{R}^n, t \in \mathbb{R}$, esto es, $g$ es $f$ pero restringida a la línea $\{x+tv|t \in \mathbb{R}\}$ , entonces:$$Dg(t) = Df(x+tv)v = \nabla f(x+tv)^Tv$$Por lo que: $$\nabla ^2g(t) = D\nabla f(x+tv)^Tv=v^T\nabla^2f(x+tv)v.$$ Ejemplo ```{margin}Ver {ref}`Ejemplo ```` Si $h: \mathbb{R}^n \rightarrow \mathbb{R}, h(x) = \log \left( \displaystyle \sum_{i=1}^m \exp(a_i^Tx+b_i)\right)$ con $x \in \mathbb{R}^n, a_i \in \mathbb{R}^n \forall i=1,\dots,m$ y $b_i \in \mathbb{R} \forall i=1,\dots,m$. Como se desarrolló anteriormente $\nabla h(x) = (1^Tz)^{-1}A^Tz$ con $z=\left[ \begin{array}{c} \exp(a_1^Tx+b_1)\\ \vdots\\ \exp(a_m^Tx+b_m) \end{array}\right]$ y $A=(a_i)_{i=1}^m \in \mathbb{R}^{m\times n}.$ Por lo que $$\nabla^2 h(x) = D\nabla h(x) = A^T \nabla^2g(Ax+b)A$$ ```{margin}$\nabla^2 g(y)$ se obtiene de acuerdo a {ref}`Caso particular ` tomando $\log:\mathbb{R} \rightarrow \mathbb{R}, \displaystyle \sum_{i=1}^m \exp(y_i): \mathbb{R}^m \rightarrow \mathbb{R}$``` donde: $\nabla^2g(y)=(1^Ty)^{-1}\text{diag}(y)-(1^Ty)^{-2}yy^T$. $$\therefore \nabla^2 h(x) = A^T\left[(1^Tz)^{-1}\text{diag}(z)-(1^Tz)^{-2}zz^T \right]A$$y $\text{diag}(c)$ es una matriz diagonal con elementos en su diagonal iguales a las entradas del vector $c$. ```{admonition} Ejercicio:class: tipVerificar con el paquete de SymPy las expresiones para la segunda derivada de los dos ejemplos anteriores.``` Tablita útil para fórmulas de diferenciación con el operador $\nabla$ Sean $f,g:\mathbb{R}^n \rightarrow \mathbb{R}$ con $f,g \in \mathcal{C}^2$ respectivamente en sus dominios y $\alpha_1, \alpha_2 \in \mathbb{R}$, $A \in \mathbb{R}^{n \times n}$, $b \in \mathbb{R}^n$ son fijas. Diferenciando con respecto a la variable $x \in \mathbb{R}^n$ se tiene: | | ||:--:|:--:||linealidad | $\nabla(\alpha_1 f(x) + \alpha_2 g(x)) = \alpha_1 \nabla f(x) + \alpha_2 \nabla g(x)$||producto | $\nabla(f(x)g(x)) = \nabla f(x) g(x) + f(x) \nabla g(x)$||producto punto|$\nabla(b^Tx) = b$ |cuadrático|$\nabla(x^TAx) = 2(A+A^T)x$||segunda derivada| $\nabla^2(Ax)=A$| Comentario respecto al cómputo simbólico o algebraico y númerico Si bien el cómputo simbólico o algebraico nos ayuda a calcular las expresiones para las derivadas evitando los problemas de errores por redondeo que se revisarán en {ref}`Polinomios de Taylor y diferenciación numérica `, la complejidad de las expresiones que internamente se manejan es ineficiente vs el cómputo numérico, ver [Computer science aspects of computer algebra](https://en.wikipedia.org/wiki/Computer_algebraComputer_science_aspects) y [GNU_Multiple_Precision_Arithmetic_Library](https://en.wikipedia.org/wiki/GNU_Multiple_Precision_Arithmetic_Library). Como ejemplo de la precisión arbitraria que se puede manejar con el cómputo simbólico o algebraico vs el {ref}`Sistema en punto flotante ` considérese el cálculo siguiente: | eps = 1-3*(4/3-1)
print("{:0.16e}".format(eps))
eps_sympy = 1-3*(sympy.Rational(4,3)-1)
print("{:0.16e}".format(float(eps_sympy))) | _____no_output_____ | Apache-2.0 | libro_optimizacion/temas/I.computo_cientifico/1.5/Definicion_de_funcion_continuidad_derivada.ipynb | vserranoc/analisis-numerico-computo-cientifico |
doit> _The use of `doit` is an implementation detail, and is subject to change!_Under the hood, the [CLI](./cli.ipynb) is powered by [doit](https://github.com/pydoit/doit), a lightweight task engine in python comparable to `make`. Using Tasks with the API | import os, pathlib, tempfile, shutil, atexit, hashlib, pandas
from IPython.display import *
from IPython import get_ipython # needed for `jupyter_execute` because magics?
import IPython
if "TMP_DIR" not in globals():
TMP_DIR = pathlib.Path(tempfile.mkdtemp(prefix="_my_lite_dir_"))
def clean():
shutil.rmtree(TMP_DIR)
atexit.register(clean)
os.chdir(TMP_DIR)
print(pathlib.Path.cwd()) | /tmp/_my_lite_dir_pskl3egv
| BSD-3-Clause | docs/doit.ipynb | marimeireles/jupyterlite |
The `LiteManager` collects all the tasks from _Addons_, and can optionally accept a `task_prefix` in case you need to integrate with existing tasks. | from jupyterlite.manager import LiteManager
manager = LiteManager(
task_prefix="lite_"
)
manager.initialize()
manager.doit_run("lite_status") | lite_static:jupyter-lite.json
. lite_pre_status:lite_static:jupyter-lite.json
tarball: jupyterlite-app-0.1.0-alpha.5.tgz 18MB
output: /tmp/_my_lite_dir_pskl3egv/_output
lite dir: /tmp/_my_lite_dir_pskl3egv
apps: ('lab', 'retro')
lite_archive:archive
lite_contents:contents
lite_lite:jupyter-lite.json
lite_serve:contents
lite_settings:overrides
. lite_status:lite_archive:archive
. lite_status:lite_contents:contents
contents: 0 files
. lite_status:lite_lite:jupyter-lite.json
. lite_status:lite_serve:contents
will serve 8000 with: tornado
. lite_status:lite_settings:overrides
overrides.json: 0
| BSD-3-Clause | docs/doit.ipynb | marimeireles/jupyterlite |
Custom Tasks and `%doit``doit` offers an IPython [magic](https://ipython.readthedocs.io/en/stable/interactive/magics.html), enabled with an extension. This can be combined to create highly reactive build tools for creating very custom sites. | %reload_ext doit | _____no_output_____ | BSD-3-Clause | docs/doit.ipynb | marimeireles/jupyterlite |
It works against the `__main__` namespace, which won't have anything by default. | %doit list | _____no_output_____ | BSD-3-Clause | docs/doit.ipynb | marimeireles/jupyterlite |
All the JupyterLite tasks can be added by updating `__main__` via `globals` | globals().update(manager._doit_tasks) | _____no_output_____ | BSD-3-Clause | docs/doit.ipynb | marimeireles/jupyterlite |
Now when a new task is created, it can reference other tasks and targets. | def task_hello():
return dict(
actions=[lambda: print("HELLO!")],
task_dep=["lite_post_status"]
)
%doit -v2 hello | lite_static:jupyter-lite.json
. lite_pre_status:lite_static:jupyter-lite.json
tarball: jupyterlite-app-0.1.0-alpha.5.tgz 18MB
output: /tmp/_my_lite_dir_pskl3egv/_output
lite dir: /tmp/_my_lite_dir_pskl3egv
apps: ('lab', 'retro')
. hello
HELLO!
| BSD-3-Clause | docs/doit.ipynb | marimeireles/jupyterlite |
Instrument Pricing Analytics - Volatility Surfaces InitialisationFirst thing I need to do is import my libraries and then run my scripts to define my helper functions. As you will note I am importing the Refinitiv Data Platform library which will be my main interface to the Platform - as well as few of the most commonly used Python libraries. | import pandas as pd
import requests
import numpy as np
import json
import refinitiv.dataplatform as rdp
%run -i c:/Refinitiv/credentials.ipynb
%run ./plotting_helper.ipynb | _____no_output_____ | Apache-2.0 | Vol Surfaces Webinar.ipynb | Refinitiv-API-Samples/Article.RDPLibrary.Python.VolatilitySurfaces_Curves |
Connect to the Refintiv Data PlatformI am using my helper functions to establish a connection the Platform by requesting a session and opening it. | session = rdp.PlatformSession(
get_app_key(),
rdp.GrantPassword(
username = get_rdp_login(),
password = get_rdp_password()
)
)
session.open() | _____no_output_____ | Apache-2.0 | Vol Surfaces Webinar.ipynb | Refinitiv-API-Samples/Article.RDPLibrary.Python.VolatilitySurfaces_Curves |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.