post_href
stringlengths 57
213
| python_solutions
stringlengths 71
22.3k
| slug
stringlengths 3
77
| post_title
stringlengths 1
100
| user
stringlengths 3
29
| upvotes
int64 -20
1.2k
| views
int64 0
60.9k
| problem_title
stringlengths 3
77
| number
int64 1
2.48k
| acceptance
float64 0.14
0.91
| difficulty
stringclasses 3
values | __index_level_0__
int64 0
34k
|
---|---|---|---|---|---|---|---|---|---|---|---|
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/2692912/Python-2-Easy-Way-To-Solve-or-95-Faster-or-Fast-and-Simple-Solution | class Solution:
def maxProfit(self, prices: List[int]) -> int:
left = 0
right = 1
maxProfit = 0
while right < len(prices):
currProfit = prices[right] - prices[left]
if prices[left] < prices[right]:
maxProfit = max(currProfit, maxProfit)
else:
left = right
right+=1
return maxProfit | best-time-to-buy-and-sell-stock | ✔️ Python 2 Easy Way To Solve | 95% Faster | Fast and Simple Solution | pniraj657 | 4 | 538 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,200 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/1645300/PYTHON-Simple-One-Pass-O(n)-solution-beats-98.66-explained | class Solution:
def maxProfit(self, prices: List[int]) -> int:
maxProfit = 0
for i in range(len(prices) - 1):
for j in range(i + 1, len(prices)):
temp = prices[j] - prices[i]
if temp > maxProfit:
maxProfit = temp
return maxProfit
# O(n^2) time
# O(1) space | best-time-to-buy-and-sell-stock | [PYTHON] Simple One Pass O(n) solution, beats 98.66%, explained | mateoruiz5171 | 4 | 607 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,201 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/695515/Python3-dp | class Solution:
def maxProfit(self, prices: List[int]) -> int:
buy, sell = inf, 0
for x in prices:
buy = min(buy, x)
sell = max(sell, x - buy)
return sell | best-time-to-buy-and-sell-stock | [Python3] dp | ye15 | 4 | 389 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,202 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/695515/Python3-dp | class Solution:
def maxProfit(self, prices: List[int]) -> int:
ans = most = 0
for i in range(1, len(prices)):
most = max(0, most + prices[i] - prices[i-1])
ans = max(ans, most)
return ans | best-time-to-buy-and-sell-stock | [Python3] dp | ye15 | 4 | 389 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,203 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/2367463/O(n)timeBEATS-99.97-MEMORYSPEED-0ms-AUG-2022 | class Solution:
def maxProfit(self,prices):
left = 0 #Buy
right = 1 #Sell
max_profit = 0
while right < len(prices):
currentProfit = prices[right] - prices[left] #our current Profit
if prices[left] < prices[right]:
max_profit =max(currentProfit,max_profit)
else:
left = right
right += 1
return max_profit | best-time-to-buy-and-sell-stock | O(n)time/BEATS 99.97% MEMORY/SPEED 0ms AUG 2022 | cucerdariancatalin | 3 | 383 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,204 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/2193558/Python-Fastest-easy-Solution | class Solution:
def maxProfit(self, prices: List[int]) -> int:
min_p = float('inf')
max_p = float('-inf')
profit = set()
for p in prices:
if p > max_p:
max_p = p
print("222 :",max_p)
if p < min_p:
min_p = p
max_p = min_p
profit.add(max_p-min_p)
return max(profit) | best-time-to-buy-and-sell-stock | Python Fastest easy Solution | vaibhav0077 | 3 | 163 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,205 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/2135452/Python-Easy-Solution-Time-Comp-O(N) | class Solution:
def maxProfit(self, prices: List[int]) -> int:
maxprofit=0
curprice = prices[0]
for i in range(1,len(prices)):
maxprofit=max(maxprofit, prices[i]-curprice)
curprice=min(curprice, prices[i])
return maxprofit | best-time-to-buy-and-sell-stock | Python Easy Solution - Time Comp O(N) | pruthashouche | 3 | 260 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,206 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/1698905/Python3-oror-easy-to-understand | class Solution:
def maxProfit(self, prices: List[int]) -> int:
mn, mx = float('inf'), 0
for i in range(len(prices)):
mn = min(prices[i], mn)
mx = max(mx, prices[i] - mn)
return mx | best-time-to-buy-and-sell-stock | Python3 || easy to understand | Anilchouhan181 | 3 | 431 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,207 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/1657293/Python-canonical-solution.-Complexity%3A-O(N)O(1) | class Solution:
def maxProfit(self, prices: List[int]) -> int:
min_ = prices[0]
max_profit = 0
for p in prices:
min_ = min(p, min_)
max_profit = max(p - min_, max_profit)
return max_profit | best-time-to-buy-and-sell-stock | Python canonical solution. Complexity: O(N)/O(1) | blue_sky5 | 3 | 242 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,208 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/1601029/Python-solution-with-easy-explanation-for-each-line-of-code | class Solution:
def maxProfit(self, prices: List[int]) -> int:
# 1.Initially the lowest price would be the first price in the given array
lowest_price = prices[0] # Accessing the first element from the given array "prices"
# And Profit would be Zero
profit = 0
# Now As we've accessed the first element from the given array we'll loop from the 2nd element upto the last last element in the array
for i in range(1,len(prices)): # looping from 2nd element upto the last element in the given array
# Check if the current price is smaller than the lowest_price
if prices[i] < lowest_price:
#If yes then make the current price as the lowest price since is the new lowest price in the given array
lowest_price = prices[i]
else: # If not then check if it maximizes the profit
profit = max(profit, prices[i] - lowest_price # compares both the values i.e profit and difference between prices[i] and lowest_price within the parenthesis and returns the maximum value
return profit #returns the profits | best-time-to-buy-and-sell-stock | Python solution with easy explanation for each line of code | pawelborkar | 3 | 387 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,209 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/946817/python3 | class Solution:
def maxProfit(self, prices: List[int]) -> int:
num_days = len(prices)
output = 0
min_price = sys.maxsize
for i in range(num_days):
if prices[i] < min_price:
min_price = prices[i]
output = max(output, prices[i] - min_price)
return output | best-time-to-buy-and-sell-stock | python3 | siyu14 | 3 | 227 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,210 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/366702/Python-linear-solution | class Solution:
def maxProfit(self, prices: List[int]) -> int:
max_profit, cur_min = 0, float('inf')
for price in prices:
cur_min = min(price, cur_min)
max_profit = max(price - cur_min, max_profit)
return max_profit | best-time-to-buy-and-sell-stock | Python linear solution | amchoukir | 3 | 577 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,211 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/2618291/SIMPLE-PYTHON3-SOLUTION-easy-to-understand-with-lineartime | class Solution:
def maxProfit(self, prices: List[int]) -> int:
maxpro = 0
n = len(prices)
minprice = prices[0]
for i in range(n):
minprice = min(minprice,prices[i])
maxpro = max(maxpro,prices[i] - minprice)
return maxpro | best-time-to-buy-and-sell-stock | ✅✔ SIMPLE PYTHON3 SOLUTION ✅✔ easy to understand with lineartime | rajukommula | 2 | 125 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,212 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/2424244/Python-simple-GRAPHICAL-explanation-or-Easy-to-understand-or-Beginners | class Solution:
def maxProfit(self, prices: List[int]) -> int:
# Step 1
low_buy = 100000 # set a very high value
high_sell = 0
#Step 2
for i in range(len(prices)):
# Step 2.1
if prices[i] < low_buy:
low_buy = prices[i]
# Step 2.2
if (prices[i] - low_buy) > high_sell:
high_sell = prices[i] - low_buy
return high_sell | best-time-to-buy-and-sell-stock | Python simple GRAPHICAL explanation | Easy to understand | Beginners | harishmanjunatheswaran | 2 | 71 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,213 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/2351965/Python-Efficient-Solution-Nice-Naming-Convention-oror-Documented | class Solution:
def maxProfit(self, prices: List[int]) -> int:
buyPrice = prices[0] # buy price at day 0
maxProfit = 0 # profit = 0, we have not sold yet.. :)
# find the sell price when have max profit: start from day 1 to last
for price in prices[1:]:
# price increased, calculate profit, if more than previous one, update it
if price > buyPrice:
maxProfit = max(maxProfit, price - buyPrice)
else:
buyPrice = price # price is dropped, set buyPrice to it
return maxProfit | best-time-to-buy-and-sell-stock | [Python] Efficient Solution Nice Naming Convention || Documented | Buntynara | 2 | 86 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,214 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/2341106/Faster-Than-99.70-or-Python-Solution | class Solution:
def maxProfit(self, prices: List[int]) -> int:
minprice = 9999999999
maxprofit = 0
for i in range(len(prices)):
if prices[i] < minprice:
minprice = prices[i]
elif (prices[i] - minprice > maxprofit):
maxprofit = prices[i] - minprice
return maxprofit | best-time-to-buy-and-sell-stock | Faster Than 99.70% | Python Solution | zip_demons | 2 | 309 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,215 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/2279038/Easy-python3-using-two-pointers | class Solution:
def maxProfit(self, prices: List[int]) -> int:
l,r = 0, 1
maxP = 0
while r < len(prices):
if prices[l] < prices[r]:
profit = prices[r] - prices[l]
maxP = max(maxP, profit)
else:
l = r
r += 1
return maxP | best-time-to-buy-and-sell-stock | Easy python3 using two pointers | __Simamina__ | 2 | 144 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,216 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/2238937/Python-Sliding-Window-Time-O(N)-or-Space-O(1)-Explained | class Solution:
def maxProfit(self, prices: List[int]) -> int:
maxProfit = 0
windowStart = 0
for windowEnd in range(len(prices)):
buyPrice = prices[windowStart]
sellPrice = prices[windowEnd]
if buyPrice > sellPrice:
windowStart = windowEnd
maxProfit = max(maxProfit, sellPrice - buyPrice)
return maxProfit | best-time-to-buy-and-sell-stock | [Python] Sliding Window - Time O(N) | Space O(1) Explained | Symbolistic | 2 | 148 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,217 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/2219558/Faster-than-96.49-of-Python3-online-submissions | class Solution:
def maxProfit(self, prices: List[int]) -> int:
n=len(prices)
max_profit=0
buy=prices[0]
for sell in range(1,n):
profit=prices[sell]-buy
max_profit=max(max_profit,profit)
buy=min(buy,prices[sell])
return max_profit | best-time-to-buy-and-sell-stock | Faster than 96.49% of Python3 online submissions | Khan_Baba | 2 | 30 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,218 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/2174124/Kadane's-Algorithm%3A-Python-Solution | class Solution:
def maxProfit(self, prices: List[int]) -> int:
maxCur = maxSoFar = 0
for i in range(1, len(prices)):
maxCur = max(0, maxCur + prices[i] - prices[i-1])
maxSoFar = max(maxCur, maxSoFar)
return maxSoFar | best-time-to-buy-and-sell-stock | Kadane's Algorithm: Python Solution | kanchitank | 2 | 227 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,219 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/1956412/Python3-Top-Down-Recursion-Memoization | class Solution:
def maxProfit(self, prices: List[int]) -> int:
@lru_cache(None)
def rec(i, k, f):
if k == 0 or i == len(prices):
return 0
c1 = rec(i+1, k, f) # we dont buy or sell
c2 = 0
if f:
c2 = rec(i+1, k-1, False) + prices[i] # we sell
else:
c2 = rec(i+1, k, True) - prices[i] # we buy
return max(c1, c2)
return rec(0, 1, False) # k == 1, means, can do only 1 transaction | best-time-to-buy-and-sell-stock | [Python3] Top Down Recursion Memoization | tushar_prajapati | 2 | 171 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,220 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/1828569/Python-solution-clear-and-simple! | class Solution:
def maxProfit(self, prices: List[int]) -> int:
maxProfit, minPrice = 0, prices[0]
for price in prices:
minPrice = min(price, minPrice)
profit = price - minPrice
maxProfit = max(profit, maxProfit)
return maxProfit | best-time-to-buy-and-sell-stock | Python solution - clear and simple! | domthedeveloper | 2 | 204 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,221 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/1736020/Python-oror-95-faster-oror-95-Space-Efficient-oror-Linear-Soln | class Solution:
def maxProfit(self, prices: List[int]) -> int:
maxi = 0
mini = 1000000
for _ in prices:
if mini > _:
mini = _
elif _ - mini > maxi:
maxi = _ - mini
return maxi | best-time-to-buy-and-sell-stock | Python || 95% faster || 95% Space Efficient || Linear Soln | cherrysri1997 | 2 | 72 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,222 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/1735950/Python3-or-beginner-friendly-or-O(n)-Solution | class Solution:
def maxProfit(self, prices: List[int]) -> int:
mn, mx = float('inf'), 0
for i in range(len(prices)):
mn= min(prices[i],mn)
mx= max(mx, prices[i]-mn)
return mx | best-time-to-buy-and-sell-stock | Python3 | beginner-friendly | O(n) Solution | Anilchouhan181 | 2 | 90 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,223 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/1735592/Python-3-EASY-Intuitive-Solution | class Solution:
def maxProfit(self, prices: List[int]) -> int:
minimum = prices[0]
profit = 0
for i in range(1, len(prices)):
if prices[i] < minimum:
minimum = prices[i]
else:
profit = max(profit, prices[i]-minimum)
return profit | best-time-to-buy-and-sell-stock | ✅ [Python 3] EASY Intuitive Solution | JawadNoor | 2 | 152 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,224 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/1681031/Simple-and-Intuitive-Python-O(n)-time-explained | class Solution:
def maxProfit(self, prices: List[int]) -> int:
minValue = pow(10,5)
maxProfit = 0
for price in prices:
if price < minValue:
minValue = price
if (price - minValue) > maxProfit:
maxProfit = price - minValue
return maxProfit | best-time-to-buy-and-sell-stock | Simple and Intuitive Python O(n) time, explained | kenanR | 2 | 310 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,225 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/1515151/Simple-Dynamic-Programming-Solution-in-Python3 | class Solution:
def maxProfit(self, prices: List[int]) -> int:
#set max profit to 0
max_profit=0
#set min price to the first price in the prices array
min_price=prices[0]
#loop from the second price in the array since first price is captured in min_price
for i in range(1,len(prices)):
#update min_price
min_price=min(min_price, prices[i])
#updte max profit
max_profit=max(max_profit,prices[i]-min_price)
return max_profit | best-time-to-buy-and-sell-stock | Simple Dynamic Programming Solution in Python3 | ninahuang2002 | 2 | 585 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,226 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/1506229/python-3-oror-easy-oror-beginner-oror-97.86-82.17 | class Solution:
def maxProfit(self, prices: List[int]) -> int:
minn=float('+inf')
profit=0
today=0
for i in range(len(prices)):
if prices[i] < minn:
minn=prices[i]
today=prices[i]-minn
if today > profit:
profit=today
return profit | best-time-to-buy-and-sell-stock | python 3 || easy || beginner || 97.86 %,82.17% | minato_namikaze | 2 | 357 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,227 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/1339895/Python-simple-sol'n-using-kadane-and-pointers-orAccepted | class Solution:
def maxProfit(self, nums: List[int]) -> int:
i = 0
j = 1
n = len(nums)
max_so_far = 0
while i<n and j<n:
if nums[i]<=nums[j]:
max_so_far = max(max_so_far,nums[j]-nums[i])
j+=1
elif nums[i]>nums[j] or j==n-1:
i+=1
j=i+1
if i==n-1:
break
return max_so_far | best-time-to-buy-and-sell-stock | [Python] simple sol'n using kadane and pointers |Accepted | pheraram | 2 | 185 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,228 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/1227318/Easy-understand-Solution-of-Python3 | class Solution:
def maxProfit(self, prices: List[int]) -> int:
length = len(prices)
if length <= 1:
return 0
max_one = 0
best_to_buy = prices[0]
for price in prices:
if price < best_to_buy:
best_to_buy = price # always buy at the lowest level
else:
max_one = max(max_one, price - best_to_buy) # try to compare every sell day
return max_one | best-time-to-buy-and-sell-stock | Easy understand Solution of Python3 | faris-shi | 2 | 101 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,229 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/2647024/How-not-to-solve-this-problem-using-Python | class Solution:
def maxProfit(self, prices: List[int]) -> int:
max_profit = []
for i in range(len(stock_val)-1):
for j in range(i+1, len(stock_val)):
if stock_val[i] < stock_val[j]:
max_profit.append(stock_val[j] - stock_val[i])
return max(max_profit)) | best-time-to-buy-and-sell-stock | How not to solve this problem using Python | code_snow | 1 | 136 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,230 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/2581079/Easy-Python-3-Solution-with-comments | class Solution:
def maxProfit(self, prices: List[int]) -> int:
max_profit = 0 #Worst case there is no profit so it stays at zero..
left = 0 #This is our buy price we want it to be less than our sell price
for right in range(left, len(prices)):
if prices[right] < prices[left]:
left = right #If not a profitable transaction then we will continue moving down the array.
else:
#See if the new transaction we just found has a higher profit than max profit
max_profit = max(max_profit, prices[right] - prices[left])
#If it the new profit is higher than the max profit then we set it to max_profit
return max_profit #Finally return the max profit! | best-time-to-buy-and-sell-stock | Easy Python 3 Solution with comments | eddie1322 | 1 | 67 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,231 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/2561632/EASY-PYTHON3-SOLUTION-two-solutions | class Solution:
def maxProfit(self, prices: List[int]) -> int:
low=10**4
max_profit=0
for i,val in enumerate(prices):
p=val-low
if val < low:
low=val
if p > max_profit:
max_profit=p
return max_profit | best-time-to-buy-and-sell-stock | ✅✔EASY PYTHON3 SOLUTION ✅✔ two solutions | rajukommula | 1 | 148 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,232 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/2561632/EASY-PYTHON3-SOLUTION-two-solutions | class Solution:
def maxProfit(self,prices):
left = 0 #Buy
right = 1 #Sell
max_profit = 0
while right < len(prices):
currentProfit = prices[right] - prices[left] #our current Profit
if prices[left] < prices[right]:
max_profit =max(currentProfit,max_profit)
else:
left = right
right += 1
return max_profit | best-time-to-buy-and-sell-stock | ✅✔EASY PYTHON3 SOLUTION ✅✔ two solutions | rajukommula | 1 | 148 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,233 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/2399585/Python3-or-Solved-Iterative-Bottom-Up-Approach-Using-Tabulation-DP | class Solution:
#Time-Complexity: O(n + n-2) -> O(n)
#Space-Complexity: O(n * 2) -> O(n)
def maxProfit(self, prices: List[int]) -> int:
if(len(prices) == 1):
return 0
first_diff = prices[1] - prices[0]
buy_price = min(prices[0], prices[1])
#dp for tabulation!
dp = [[] for _ in range(len(prices))]
#if we consider up to first 2 days, we already know max revenue which is just
#difference of first two days' prices as well as the optimal buy price!
dp[1].append(first_diff)
dp[1].append(buy_price)
#bottom-up approach! -> Finding max profit i can get if I consider each and every
#additional ith day I did not take account in prev. iteration!
#Basically, checking if current day price if i sell on minus the lowest buy price
#in previous time frame could potentially beat the already computed max profit
#of using prices up to i-1th day!
for i in range(2, len(prices)):
dp[i].append(max(dp[i-1][0], prices[i] - dp[i-1][1]))
dp[i].append(min(dp[i-1][1], prices[i]))
#now once we are done with for loop solving for finding
#max profit if we consider subset of prices up to 3rd day or
#all the way up to last day!
#we get the last dp array's first element! -> If the best profit we could do is negative,
#then return 0 as it is rather a loss!
return dp[-1][0] if dp[-1][0] > 0 else 0 | best-time-to-buy-and-sell-stock | Python3 | Solved Iterative Bottom-Up Approach Using Tabulation DP | JOON1234 | 1 | 74 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,234 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/2329066/O(N)-Approach-for-C%2B%2BPython-best-optimized-one | class Solution:
def maxProfit(self, prices: List[int]) -> int:
minPrice = 9999999999
maxProfit = 0
for i in range(len(prices)):
profit = prices[i] - minPrice
minPrice = min(minPrice, prices[i])
maxProfit = max(maxProfit, profit)
return maxProfit | best-time-to-buy-and-sell-stock | O(N) Approach for C++/Python best optimized one | arpit3043 | 1 | 59 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,235 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/2297079/Simple-python3-using-two-pointers(updated) | class Solution:
def maxProfit(self, prices: List[int]) -> int:
l,r = 0, 1
maxP = 0
while r < len(prices):
if prices[l] < prices[r]:
profit = prices[r] - prices[l]
maxP = max(maxP, profit)
else:
l = r
r += 1
return maxP | best-time-to-buy-and-sell-stock | Simple python3 using two pointers(updated) | __Simamina__ | 1 | 89 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,236 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/2156028/Python-solution | class Solution:
def maxProfit(self, prices: List[int]) -> int:
res = 0 # Initializing result to 0.
buy = prices[0] # Buy is intialized to the first element
for sell in prices: # Looping in the prices list
if(buy<sell): # Comparing if first element is less than any other element in the list.
profit = sell - buy # If yes, then profit would be sell-buy.
res = max(res, profit) # And we'll calculate the result as maximum of result and profit.
else: # If not, then buy would be equal to sell, so now buy would be reinitialized.
buy = sell
return res # We'll return the result as res. | best-time-to-buy-and-sell-stock | Python solution | yashkumarjha | 1 | 88 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,237 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/2086701/Python3-Clean-and-Fast | class Solution:
# O(n) time | O(1) space
def maxProfit(self, prices: List[int]) -> int:
max_profit, min_price = 0, float("inf")
for i, price in enumerate(prices):
max_profit = max(max_profit, price - min_price)
min_price = min(min_price, price)
return max_profit | best-time-to-buy-and-sell-stock | Python3 - Clean and Fast | ig051 | 1 | 156 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,238 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/2075714/Python3-its-similar-to-Kadane's-algorithm | class Solution:
def maxProfit(self, prices: List[int]) -> int:
return self.maxProfitOptimal(prices)
# return self.maxProfitByTwoLoops(prices)
# O(n) || O(1) 1778ms 15.94%
def maxProfitOptimal(self, prices):
if not prices:
return 0
minVal = prices[0]
maxVal = float('-inf')
for i in range(1, len(prices)):
profit = prices[i] - minVal
maxVal = max(maxVal, profit)
minVal = min(minVal, prices[i])
return maxVal if maxVal > 0 else 0
# O(N^2) || O(1) TLE
def maxProfitByTwoLoops(self, prices):
if not prices:
return prices
maxVal = float('-inf')
for i in range(len(prices)):
for j in range(i+1, len(prices)):
maxVal = max(maxVal, prices[j] - prices[i])
return maxVal if maxVal > 0 else 0 | best-time-to-buy-and-sell-stock | Python3 its similar to Kadane's algorithm | arshergon | 1 | 94 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,239 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/2058036/Python-or-Recursion | class Solution:
def maxProfit(self, pr: List[int]) -> int:
def solve(i,j):
if i==j:
return 0
if i>j:
return -1
mid = (i+j)//2
# print(i,mid,j)
t = min(pr[i:mid+1])
t1 = max(pr[mid+1:j+1])
if t1-t>=0:
return max(t1-t,solve(i,mid),solve(mid+1,j))
else:
return max(solve(i,mid),solve(mid+1,j))
return solve(0,len(pr) - 1) | best-time-to-buy-and-sell-stock | Python | Recursion | Shivamk09 | 1 | 185 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,240 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/2023726/Python-easy-O(n)-solution | class Solution:
def maxProfit(self, prices: List[int]) -> int:
buy_price = sys.maxsize
max_profit = 0
for current_price in prices:
if current_price < buy_price:
buy_price = current_price
else:
max_profit = max(max_profit, current_price - buy_price)
return max_profit | best-time-to-buy-and-sell-stock | Python easy O(n) solution | AlexChantsev | 1 | 194 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,241 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/1947996/Super-simple-answer-in-python.-8588 | class Solution:
def maxProfit(self, prices: List[int]) -> int:
max_gain = 0
min_price = prices[0]
for current_price in prices[1:]:
current_gain = current_price - min_price #calculate current gain
max_gain = max(max_gain, current_gain) #update max gain
min_price = min(min_price, current_price) #update min price
return max_gain | best-time-to-buy-and-sell-stock | Super simple answer in python. 85%/88% | user4553BE | 1 | 63 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,242 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/1713935/simple-python-solution-0(n) | class Solution:
def maxProfit(self, prices: List[int]) -> int:
#prices = [7,1,5,3,6,4]
right_side=1
max_pr = 0
size = len(prices)
buy_price = prices[0] # 7
while right_side < size:
if buy_price <= prices[right_side]:
diff = prices[right_side] - buy_price
max_pr = max(max_pr, diff )
else:
buy_price = prices[right_side]
right_side+=1
return max_pr | best-time-to-buy-and-sell-stock | simple python solution 0(n) | suman2020 | 1 | 151 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,243 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/1671779/python-Iteration-%2B-variable | class Solution:
def maxProfit(self, prices: List[int]) -> int:
# Time:O(n), Space: O(1)
lowest = prices[0]
maxvalue = 0
for i in range(1,len(prices)):
maxvalue = max(maxvalue, prices[i]-lowest)
lowest = min(lowest,prices[i])
return maxvalue | best-time-to-buy-and-sell-stock | [python] Iteration + variable | JackYeh17 | 1 | 97 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,244 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/1598519/Python3-One-pass-O(1)-space-solution | class Solution:
def maxProfit(self, prices: List[int]) -> int:
_min_price = float('inf')
res = 0
for price in prices:
if price < _min_price:
_min_price = price
else:
res = max(res, price - _min_price)
return res | best-time-to-buy-and-sell-stock | [Python3] One pass, O(1) space solution | maosipov11 | 1 | 128 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,245 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock/discuss/1589431/Python3-one-pass-O(n)-time-and-O(1)-space | class Solution:
def maxProfit(self, prices: List[int]) -> int:
min_price, max_profit = math.inf, 0
for p in prices:
if p < min_price:
min_price = p
elif p-min_price > max_profit:
max_profit = p-min_price
return max_profit | best-time-to-buy-and-sell-stock | [Python3] one-pass O(n) time & O(1) space | JosephJia | 1 | 251 | best time to buy and sell stock | 121 | 0.544 | Easy | 1,246 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2040292/O(n)timeBEATS-99.97-MEMORYSPEED-0ms-MAY-2022 | class Solution:
def maxProfit(self, prices: List[int]) -> int:
@cache
def trade(day_d):
if day_d == 0:
# Hold on day_#0 = buy stock at the price of day_#0
# Not-hold on day_#0 = doing nothing on day_#0
return -prices[day_d], 0
prev_hold, prev_not_hold = trade(day_d-1)
hold = max(prev_hold, prev_not_hold - prices[day_d] )
not_hold = max(prev_not_hold, prev_hold + prices[day_d] )
return hold, not_hold
# --------------------------------------------------
last_day= len(prices)-1
# Max profit must come from not_hold state (i.e., no stock position) on last day
return trade(last_day)[1] | best-time-to-buy-and-sell-stock-ii | O(n)time/BEATS 99.97% MEMORY/SPEED 0ms MAY 2022 | cucerdariancatalin | 12 | 605 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,247 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2434293/Easy-oror-100-oror-4-Line-oror-Fully-Explained-(Java-C%2B%2B-Python-JS-C-Python3) | class Solution(object):
def maxProfit(self, prices):
# Initialize the max profit...
maximumProfit = 0
# Traverse all the element through loop...
for i in range(1, len(prices)):
# check if the price is greater at i...
if prices[i] > prices[i-1]:
# Add the difference to profit and increse it...
maximumProfit += prices[i] - prices[i-1]
return maximumProfit # Return the max profit... | best-time-to-buy-and-sell-stock-ii | Easy || 100% || 4 Line || Fully Explained (Java, C++, Python, JS, C, Python3) | PratikSen07 | 7 | 430 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,248 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2434293/Easy-oror-100-oror-4-Line-oror-Fully-Explained-(Java-C%2B%2B-Python-JS-C-Python3) | class Solution:
def maxProfit(self, prices: List[int]) -> int:
# Initialize the max profit...
maximumProfit = 0
# Traverse all the element through loop...
for i in range(1, len(prices)):
# check if the price is greater at i...
if prices[i] > prices[i-1]:
# Add the difference to profit and increse it...
maximumProfit += prices[i] - prices[i-1]
return maximumProfit # Return the max profit... | best-time-to-buy-and-sell-stock-ii | Easy || 100% || 4 Line || Fully Explained (Java, C++, Python, JS, C, Python3) | PratikSen07 | 7 | 430 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,249 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/565654/Python3-greedy-and-dp | class Solution:
def maxProfit(self, prices: List[int]) -> int:
return sum(max(0, prices[i] - prices[i-1]) for i in range(1, len(prices))) | best-time-to-buy-and-sell-stock-ii | [Python3] greedy & dp | ye15 | 4 | 241 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,250 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/565654/Python3-greedy-and-dp | class Solution:
def maxProfit(self, prices: List[int]) -> int:
ans = 0
for i in range(1, len(prices)):
ans += max(0, prices[i] - prices[i-1])
return ans | best-time-to-buy-and-sell-stock-ii | [Python3] greedy & dp | ye15 | 4 | 241 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,251 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/565654/Python3-greedy-and-dp | class Solution:
def maxProfit(self, prices: List[int]) -> int:
buy, sell = [0]*len(prices), [0]*len(prices)
buy[0] = prices[0]
for i in range(1, len(prices)):
buy[i] = min(buy[i-1], prices[i] - sell[i-1])
sell[i] = max(sell[i-1], prices[i] - buy[i])
return sell[-1] | best-time-to-buy-and-sell-stock-ii | [Python3] greedy & dp | ye15 | 4 | 241 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,252 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/565654/Python3-greedy-and-dp | class Solution:
def maxProfit(self, prices: List[int]) -> int:
buy, sell = inf, 0
for x in prices:
buy = min(buy, x - sell)
sell = max(sell, x - buy)
return sell | best-time-to-buy-and-sell-stock-ii | [Python3] greedy & dp | ye15 | 4 | 241 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,253 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/565654/Python3-greedy-and-dp | class Solution:
def maxProfit(self, prices: List[int]) -> int:
ans = most = 0
for i in range(1, len(prices)):
most = max(0, prices[i] - prices[i-1])
ans += most
return ans | best-time-to-buy-and-sell-stock-ii | [Python3] greedy & dp | ye15 | 4 | 241 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,254 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/1116903/WEEB-DOES-PYTHON-FOR-YOU | class Solution:
def maxProfit(self, prices: List[int]) -> int:
profit = 0
for i in range(len(prices)-1):
if prices[i] < prices[i+1]:
profit += prices[i+1] - prices[i]
return profit | best-time-to-buy-and-sell-stock-ii | WEEB DOES PYTHON FOR YOU | Skywalker5423 | 3 | 319 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,255 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2797655/Linear-Order-Python-Solution | class Solution:
def maxProfit(self, prices: List[int]) -> int:
n=len(prices)
buy=0
dp=[[-1]*2 for i in range(n+1)]
(dp[n])[0]=(dp[n])[0]=0
for ind in range(n-1,-1,-1):
for buy in range(0,2):
if buy==0:
(dp[ind])[buy]=max((-prices[ind]+(dp[ind+1])[1]),(dp[ind+1])[0])
else:
(dp[ind])[buy]=max((+prices[ind]+(dp[ind+1])[0]),(dp[ind+1])[1])
return (dp[0])[0] | best-time-to-buy-and-sell-stock-ii | Linear Order Python Solution | Khan_Baba | 2 | 373 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,256 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2113567/Python-or-4-Approaches-or-Entire-DP-or | class Solution:
def maxProfit(self, prices: List[int]) -> int:
n = len(prices)
dp = [[-1 for i in range(2)] for i in range(n+1)]
dp[n][0] = dp[n][1] = 0
ind = n-1
while(ind>=0):
for buy in range(2):
if(buy):
profit = max(-prices[ind] + dp[ind+1][0], 0 + dp[ind+1][1])
else:
profit = max(prices[ind] + dp[ind+1][1], 0 + dp[ind+1][0])
dp[ind][buy] = profit
ind -= 1
return dp[0][1] | best-time-to-buy-and-sell-stock-ii | Python | 4 Approaches | Entire DP | | LittleMonster23 | 2 | 150 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,257 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2113567/Python-or-4-Approaches-or-Entire-DP-or | class Solution:
def maxProfit(self, prices: List[int]) -> int:
n = len(prices)
ahead = [0 for i in range(2)]
curr = [0 for i in range(2)]
ahead[0] = ahead[1] = 0
ind = n-1
while(ind>=0):
for buy in range(2):
if(buy):
profit = max(-prices[ind] + ahead[0], 0 + ahead[1])
else:
profit = max(prices[ind] + ahead[1], 0 + ahead[0])
curr[buy] = profit
ahead = [x for x in curr]
ind -= 1
return ahead[1] | best-time-to-buy-and-sell-stock-ii | Python | 4 Approaches | Entire DP | | LittleMonster23 | 2 | 150 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,258 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/337075/Python-simple-solution-O(n) | class Solution:
def maxProfit(self, prices: List[int]) -> int:
maxpro=0
if not prices:return 0
for i in range(len(prices)-1):
if prices[i]<prices[i+1]:
maxpro+=prices[i+1]-prices[i]
return maxpro | best-time-to-buy-and-sell-stock-ii | Python simple solution O(n) | ketan35 | 2 | 331 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,259 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/255013/Python-Easy-to-Understand | class Solution:
def maxProfit(self, prices: List[int]) -> int:
maxProfit = 0
for i in range(1, len(prices)):
if prices[i] > prices[i-1]:
maxProfit += prices[i] - prices[i-1]
return maxProfit | best-time-to-buy-and-sell-stock-ii | Python Easy to Understand | ccparamecium | 2 | 284 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,260 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2816169/One-pass-straightforward-or-SC%3A-O(N)-TC%3A-O(1) | class Solution:
def maxProfit(self, prices: List[int]) -> int:
# Buy very first stock
buy = prices[0]
profit = 0
for i in range(1, len(prices)):
# If you find stock less than what you bought, take that instead
if prices[i] < buy:
buy = prices[i]
# If you find stock greater than what you bought, sell that
# Also buy it as you want to sell if you see greater stock in future
else:
profit += prices[i] - buy
buy = prices[i]
return profit | best-time-to-buy-and-sell-stock-ii | One-pass, straightforward | SC: O(N), TC: O(1) | bikcrum | 1 | 187 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,261 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2558060/Python3-Solution-or-Sliding-Window-or-Beats-97-Time | class Solution:
def maxProfit(self, prices: List[int]) -> int:
total_profit = 0
i = 0
j = 0
while j < len(prices):
if prices[j] <= prices[i]:
i = j
else:
while j < len(prices)-1 and prices[j+1] > prices[j]:
j += 1
profit = prices[j] - prices[i]
total_profit += profit
i = j + 1
j += 1
return total_profit | best-time-to-buy-and-sell-stock-ii | Python3 Solution | Sliding Window | Beats 97% Time | dos_77 | 1 | 10 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,262 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2429685/Python-DP-Solution-(Memoization) | class Solution:
def maxProfit(self, prices: List[int]) -> int:
d = {}
def bs(index, buyOrNotBuy):
if index >= len(prices):
return 0
# if we are able to buy the stock, if we already sold it before or
# if we have not bought any stock
if buyOrNotBuy == "buy":
# if buy stock at this index
if (index+1, "notBuy") not in d:
profit = -1 * prices[index] + bs(index+1, "notBuy")
else:
profit = -1 * prices[index] + d[(index+1, "notBuy")]
# if buy later, not now at this index
if (index+1, "buy") not in d:
profit1 = bs(index+1, "buy")
else:
profit1 = d[(index+1, "buy")]
d[(index, buyOrNotBuy)] = max(profit, profit1)
return d[(index, buyOrNotBuy)]
else:
# sell stock, if we sell 2nd argument is buy
# sell stock at this index
if (index+1, "buy") not in d:
profit = prices[index] + bs(index+1, "buy")
else:
profit = prices[index] + d[(index+1, "buy")]
# sell stock not at this index now, sell it later
if (index+1, "notBuy") not in d:
profit1 = bs(index+1, "notBuy")
else:
profit1 = d[(index+1, "notBuy")]
d[(index, buyOrNotBuy)] = max(profit, profit1)
return d[(index, buyOrNotBuy)]
return bs(0, "buy") | best-time-to-buy-and-sell-stock-ii | Python DP Solution (Memoization) | DietCoke777 | 1 | 53 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,263 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2398678/Python-91.76-faster-or-Simplest-solution-with-explanation-or-Beg-to-Adv-or-Greedy | class Solution:
def maxProfit(self, prices: List[int]) -> int:
profit = 0 # to store the total profit
for i in range(1, len(prices)): # traversing the prices list, taking it from 1st index so that we could compare the day gain or loss by comparing it to previous value.
if prices[i]>prices[i-1]: # comparing current value with last value
profit += (prices[i] - prices[i-1]) # if we have a great value implies we have profit. But we need total profit means after removing losses thats why we are subtracting previous value which is low.
return profit # returning total profit earned. | best-time-to-buy-and-sell-stock-ii | Python 91.76% faster | Simplest solution with explanation | Beg to Adv | Greedy | rlakshay14 | 1 | 71 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,264 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2060124/Python-or-Easy-Understanding | class Solution:
def maxProfit(self, pr: List[int]) -> int:
ans = 0
for i in range(len(pr)):
if i==0:
mi = pr[i]
else:
if pr[i]<pr[i-1]:
ans += pr[i-1]-mi
mi = pr[i]
ans += pr[i]-mi
return ans | best-time-to-buy-and-sell-stock-ii | Python | Easy Understanding | Shivamk09 | 1 | 50 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,265 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/1792067/Concise-Code-or-Python | class Solution:
def maxProfit(self, prices: List[int]) -> int:
tot_profit = 0
buy_price = prices[0]
for i in range(1,len(prices)):
if prices[i] > buy_price:
tot_profit += prices[i] - buy_price
buy_price = prices[i]
return tot_profit | best-time-to-buy-and-sell-stock-ii | Concise Code | Python | iamskd03 | 1 | 63 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,266 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/1782181/Python-easy-to-read-and-understand-or-DP | class Solution:
def maxProfit(self, prices: List[int]) -> int:
profit, buy = 0, prices[0]
for i in range(1, len(prices)):
if prices[i] > prices[i-1]:
profit += (prices[i]-buy)
buy = prices[i]
else:
buy = prices[i]
return profit | best-time-to-buy-and-sell-stock-ii | Python easy to read and understand | DP | sanial2001 | 1 | 172 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,267 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/1564442/Python3-Solution-or-O(n) | class Solution:
def maxProfit(self, prices: List[int]) -> int:
profit = 0
for i in range(1,len(prices)):
if prices[i] > prices[i-1]:
profit += prices[i]-prices[i-1]
return profit | best-time-to-buy-and-sell-stock-ii | Python3 Solution | O(n) | satyam2001 | 1 | 116 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,268 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/1564442/Python3-Solution-or-O(n) | class Solution:
def maxProfit(self, prices: List[int]) -> int:
profit = 0
for i in range(1,len(prices)):
profit += max(prices[i]-prices[i-1],0)
return profit | best-time-to-buy-and-sell-stock-ii | Python3 Solution | O(n) | satyam2001 | 1 | 116 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,269 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/1522537/O(n)-with-O(1)-space. | class Solution:
def maxProfit(self, prices: List[int]) -> int:
profit = 0
for x in range(1,len(prices)):
profit+=max(0, prices[x]-prices[x-1])
return profit | best-time-to-buy-and-sell-stock-ii | O(n), with O(1) space. | user7797Mu | 1 | 81 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,270 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/1441331/Python-3-Solution-(DO-NOT-MAKE-THIS-MISTAKE) | class Solution:
def maxProfit(self, prices: List[int]) -> int:
def strictly_increasing(buy_d,arr):
for i in range(buy_d-1,len(arr)-1):
if arr[i] < arr[i+1]:
continue
else:
return i
return len(arr)-1
a = 0
max_profit = 0
while a < len(prices)-1:
buy_price = prices[a]
sell_price = prices[strictly_increasing(a+1,prices)]
profit = sell_price - buy_price
max_profit += profit
a = strictly_increasing(a+1,prices) + 1
return max_profit | best-time-to-buy-and-sell-stock-ii | Python 3 Solution (DO NOT MAKE THIS MISTAKE) | deleted_user | 1 | 98 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,271 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/1441331/Python-3-Solution-(DO-NOT-MAKE-THIS-MISTAKE) | class Solution:
def maxProfit(self, prices: List[int]) -> int:
profit = 0
for i in range(1, len(prices)):
profit += max(0, prices[i] - prices[i-1])
return profit | best-time-to-buy-and-sell-stock-ii | Python 3 Solution (DO NOT MAKE THIS MISTAKE) | deleted_user | 1 | 98 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,272 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/1370396/O(n)-97.96-faster-Python-Simple-solution | class Solution:
def maxProfit(self, prices: List[int]) -> int:
profit = 0
for i in range(1,len(prices)):
if(prices[i]>prices[i-1]):
profit+=prices[i]-prices[i-1]
return profit
```
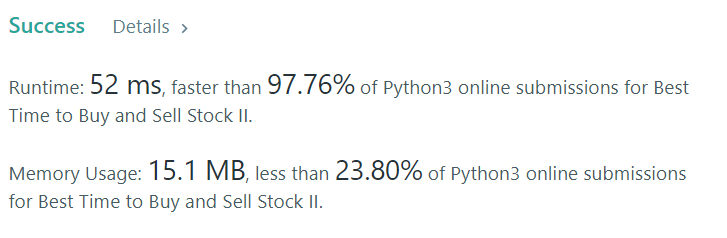 | best-time-to-buy-and-sell-stock-ii | O(n), 97.96% faster, Python, Simple solution | unKNOWN-G | 1 | 331 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,273 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/1065440/python | class Solution:
def maxProfit(self, prices: List[int]) -> int:
minprice = prices[0]
profit = 0
for i in prices:
if i > minprice:
profit += i - minprice
minprice = i
return profit | best-time-to-buy-and-sell-stock-ii | python | nancyszy | 1 | 132 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,274 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/617637/Intuitive-approach-with-intuitive-buyingselling-strategy | class Solution:
def maxProfit(self, prices: List[int]) -> int:
pp = prices[0]
r'''
* Buy at point where next point will rise
* Sell at point where next point will drop
'''
sum_of_earned = 0
''' Total earned from selling stock'''
buy_price = -1
''' Used to keep the price of stock being bought'''
prices_size = len(prices)
''' Length of price array '''
for i in range(len(prices)-1):
now_p = prices[i] # Current price
next_p = prices[i+1] # Tomorrow's price
if next_p < now_p:
# stock will drop tomorrow
if buy_price >= 0:
sum_of_earned += (now_p - buy_price)
buy_price = -1
elif next_p > now_p:
# stock will rise tomorrow
if buy_price < 0:
buy_price = now_p
if buy_price >= 0 and prices[-1] > buy_price:
# We still have stock in hand
# Sell it if the price is higher than the price we bought
sum_of_earned += (prices[-1] - buy_price)
return sum_of_earned | best-time-to-buy-and-sell-stock-ii | Intuitive approach with intuitive buying/selling strategy | puremonkey2001 | 1 | 77 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,275 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/268595/Python-40ms | class Solution:
def maxProfit(self, prices: List[int]) -> int:
total = 0
for day in range(len(prices)-1):
if prices[day] < prices[day+1]:
total += prices[day+1] - prices[day]
return total | best-time-to-buy-and-sell-stock-ii | Python 40ms | mobiplayer | 1 | 115 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,276 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2845477/Easy-to-understand-beats-75 | class Solution:
def maxProfit(self, prices: List[int]) -> int:
dp=[0]*len(prices)
for i in range(0,len(prices)-1,1):
for j in range(i+1,i+2):
if(max(prices[i],prices[j]))==prices[j]:
dp[i]=prices[j]-prices[i]
else:
pass
return sum(dp) | best-time-to-buy-and-sell-stock-ii | Easy to understand , beats 75% | varunmuthannaka | 0 | 2 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,277 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2839408/Surprisingly-easy-to-understand-O(n)-solution-in-Python | class Solution:
def maxProfit(self, prices: List[int]) -> int:
diff = []
profit = 0
for i in range(1, len(prices)):
diff.append(prices[i] - prices[i - 1])
for d in diff:
profit = profit + d if d > 0 else profit
return profit | best-time-to-buy-and-sell-stock-ii | Surprisingly easy to understand O(n) solution in Python | Whateverokokokok | 0 | 2 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,278 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2839408/Surprisingly-easy-to-understand-O(n)-solution-in-Python | class Solution:
def maxProfit(self, prices: List[int]) -> int:
profit = 0
for i in range(1, len(prices)):
diff = prices[i] - prices[i - 1]
profit = profit + diff if diff >0 else profit
return profit | best-time-to-buy-and-sell-stock-ii | Surprisingly easy to understand O(n) solution in Python | Whateverokokokok | 0 | 2 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,279 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2837612/Python-90-faster | class Solution:
def maxProfit(self, prices: List[int]) -> int:
pft = 0
i = 0
while i + 1 < len(prices):
diff = prices[i + 1] - prices[i]
if diff > 0:
pft += diff
i += 1
return pft | best-time-to-buy-and-sell-stock-ii | Python = 90% faster | fatalbanana | 0 | 4 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,280 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2825831/o(n)-Python | class Solution:
def maxProfit(self, prices: List[int]) -> int:
profit_ = 0
buy = float("inf")
for i in range(len(prices)):
if prices[i] < buy:
buy = prices[i]
elif prices[i] - buy > 0:
profit_ += prices [i] - buy
buy = prices[i]
return profit_ | best-time-to-buy-and-sell-stock-ii | o(n) Python | AkshayVijayBidwai | 0 | 3 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,281 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2823401/O(n)-time-complexity-Python-easy-solution | class Solution:
def maxProfit(self, prices: List[int]) -> int:
mini,maxi = prices[0],-1
cost = 0
for val in prices:
if val < mini:
mini = val
if val > mini:
maxi = val
cost += maxi-mini
mini = val
maxi = -1
return cost | best-time-to-buy-and-sell-stock-ii | O(n) time complexity - Python easy solution | vasanth180799 | 0 | 2 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,282 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2820825/Optimized-and-Easy-Solution | class Solution:
def maxProfit(self, prices: List[int]) -> int:
profit = 0
for i in range(1, len(prices)):
if prices[i] > prices[i - 1]:
profit += (prices[i] - prices[i - 1])
return profit | best-time-to-buy-and-sell-stock-ii | Optimized and Easy Solution | swaruptech | 0 | 1 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,283 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2816135/Python-Solution | class Solution:
def maxProfit(self, prices: List[int]) -> int:
p = 0
b = prices[0]
l = prices[0]
for idx, i in enumerate(prices):
if i < l:
p += (l - b)
b = i
l = i
else:
l = i
p += (l - b)
return p | best-time-to-buy-and-sell-stock-ii | Python Solution | jaisalShah | 0 | 1 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,284 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2792622/Best-Time-to-Buy-and-to-Sell-Stock-II-(Python-optimal-solution) | class Solution:
def maxProfit(self, prices: List[int]) -> int:
profit = 0
for i in range(1, len(prices)):
if prices[i] > prices[i-1]:
# sell
profit += prices[i] - prices[i-1]
return profit | best-time-to-buy-and-sell-stock-ii | Best Time to Buy and to Sell Stock II (Python optimal solution) | riya_gupta28 | 0 | 1 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,285 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2785091/40-faster-Python-Code-TC%3AO(N)-and-SC%3AO(1) | class Solution:
def maxProfit(self, prices: List[int]) -> int:
maxProfit = 0
for i in range(1,len(prices)):
if prices[i] - prices[i-1] > 0:
maxProfit += prices[i] - prices[i-1]
return maxProfit | best-time-to-buy-and-sell-stock-ii | 40% faster Python Code TC:O(N) and SC:O(1) | sudharshan1706 | 0 | 3 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,286 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2776415/Greedy-simple-modification-from-Best-Time-to-Buy-and-Sell-Stock-I | class Solution:
def maxProfit(self, prices: List[int]) -> int:
if len(prices) < 2:
return 0
resultList = []
MaxProfit = 0
CurrVal = prices[0]
for i in range(len(prices)):
if prices[i] > CurrVal:
MaxProfit += (prices[i] - CurrVal) #Sell the stock
CurrVal = prices[i]
if prices[i] < CurrVal:
CurrVal = prices[i]
return MaxProfit | best-time-to-buy-and-sell-stock-ii | Greedy, simple modification from Best Time to Buy and Sell Stock I | ZihanWang1819 | 0 | 1 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,287 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2775302/Python-Easy-solution-O(n) | class Solution:
def maxProfit(self, prices: List[int]) -> int:
holding = False
day = 0
N = len(prices)
buy = 0
profit = 0
while day < N-1:
if not holding:
if prices[day] > prices[day+1]:
pass
else:
buy = prices[day]
holding = True
else:
if prices[day+1] > prices[day] and prices[day] >= buy:
pass
else:
profit += prices[day] - buy
holding = False
day += 1
if holding:
sale = prices[day] - buy
if sale > 0:
profit += sale
return profit | best-time-to-buy-and-sell-stock-ii | [Python] Easy solution O(n) | koff82 | 0 | 5 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,288 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2767533/Python-four-approach-very-easy | class Solution:
def solve(self , i , buy , prices , n):
if i >= n:
return 0
if buy == 0:
no = 0 + self.solve(i+1 , 0 , prices , n)
take = -prices[i] + self.solve(i+1 , 1 , prices , n)
profit = max( take , no)
if buy == 1:
profit = max(0 + self.solve(i+1 , 1 , prices , n) , prices[i] + self.solve(i+1 , 0 , prices , n))
return profit
def maxProfit(self, prices: List[int]) -> int:
n = len(prices)
return self.solve(0 , 0 , prices , n)
""" memorization """
class Solution:
def solve(self , i , buy , prices , n , memo):
if i >= n:
return 0
if memo[i][buy] != -1:
return memo[i][buy]
if buy == 0:
no = 0 + self.solve(i+1 , 0 , prices , n , memo)
take = -prices[i] + self.solve(i+1 , 1 , prices , n , memo)
profit = max( take , no)
memo[i][buy] = profit
if buy == 1:
profit = max(0 + self.solve(i+1 , 1 , prices , n , memo) , prices[i] + self.solve(i+1 , 0 , prices , n , memo))
memo[i][buy] = profit
memo[i][buy] = profit
return memo[i][buy]
def maxProfit(self, prices: List[int]) -> int:
n = len(prices)
memo = [[-1 for j in range(2)] for i in range(n)]
return self.solve(0 , 0 , prices , n , memo)
""" Bottom up """
class Solution:
def maxProfit(self, prices: List[int]) -> int:
n = len(prices)
dp = [[0 for j in range(2)] for i in range(n+1)]
dp[n][0] = dp[n][1] = 0
for i in range(n-1 , -1 , -1):
for j in range(2):
if j == 0:
no = 0 + dp[i+1][0]
take = -prices[i] + dp[i+1][1]
profit = max(no , take)
dp[i][j] = profit
if j == 1:
no = 0 + dp[i+1][1]
take = prices[i] + dp[i+1][0]
profit = max(no , take)
dp[i][j] = profit
return dp[0][0]
""" my fav solution """
class Solution:
def maxProfit(self, prices: List[int]) -> int:
profit = 0
cost = prices[0]
for i in range(1 , len(prices)):
if prices[i] > cost:
profit += prices[i] - cost
cost = prices[i]
return profit | best-time-to-buy-and-sell-stock-ii | Python four approach very easy | rajitkumarchauhan99 | 0 | 2 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,289 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2760390/Easiest-Python-Solution-you-are-looking-for! | class Solution:
def maxProfit(self, prices: List[int]) -> int:
minimum=+math.inf
profit=0
for i in prices:
minimum=min(minimum,i)
if i>minimum:
profit+=i-minimum
minimum=i
return profit | best-time-to-buy-and-sell-stock-ii | Easiest Python Solution you are looking for! | Nikhil_Namburi | 0 | 3 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,290 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2754672/Simple-Iterative-Approach-or-Python | class Solution:
def maxProfit(self, prices: List[int]) -> int:
ans, res = 0, prices[0]
n = len(prices)
for i in range(1,n):
if prices[i]>res:
ans += prices[i]-res
res = prices[i]
else:
res = prices[i]
return ans | best-time-to-buy-and-sell-stock-ii | Simple Iterative Approach | Python | RajatGanguly | 0 | 3 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,291 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2734321/better-than-97.5-percentage-PYTHON | class Solution:
def maxProfit(self, prices: List[int]) -> int:
buy = prices[0]
maxProfit = 0
for i in range(1,len(prices)):
if buy > prices[i]:
buy = prices[i]
elif 0 < prices[i] - buy:
maxProfit += prices[i] - buy
buy = prices[i]
return maxProfit | best-time-to-buy-and-sell-stock-ii | better than 97.5 percentage PYTHON | Taksh_beladiya | 0 | 6 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,292 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2732895/Fast-and-Easy-Solution | class Solution:
def maxProfit(self, prices: List[int]) -> int:
buying_price = prices[0]
max_profit = 0
for selling_price in prices[1 : ]:
if buying_price > selling_price:
buying_price = selling_price
else:
max_profit += (selling_price - buying_price)
buying_price = selling_price
return max_profit | best-time-to-buy-and-sell-stock-ii | Fast and Easy Solution | user6770yv | 0 | 4 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,293 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2730129/same-as-leetcode-1911-easy-dp | class Solution:
def maxProfit(self, prices: List[int]) -> int:
even = 0
odd = 0
for num in prices[::-1] :
temp_even = even
even = max(even, odd + num)
odd = max(odd, temp_even - num)
return odd
#def maxAlternatingSum(self, nums: List[int]) -> int:
#even = 0
#odd = 0
#for num in nums :
# even = max(even, odd + num)
# odd = max(odd, even - num)
#return even | best-time-to-buy-and-sell-stock-ii | same as leetcode 1911, easy dp | user8498Sr | 0 | 3 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,294 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2683018/Python-solution-beats-99-users | class Solution:
def maxProfit(self, prices: List[int]) -> int:
p=prices
return sum([max(0,p[i]-p[i-1]) for i in range(1,len(p))]) | best-time-to-buy-and-sell-stock-ii | Python solution beats 99% users | mritunjayyy | 0 | 4 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,295 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2681512/Positive-Pair-Difference-Approach-oror-Intuitive-oror-Beats-92 | class Solution:
def maxProfit(self, prices: List[int]) -> int:
if len(prices) <= 1:
return 0
totalProfit = 0
for i in range(1,len(prices)):
totalProfit +=
prices[i]-prices[i-1] if prices[i]-prices[i-1] > 0 else 0
return totalProfit | best-time-to-buy-and-sell-stock-ii | Positive Pair Difference Approach || Intuitive || Beats 92% | mayankleetcode | 0 | 1 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,296 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2653158/Easy-Understandable-Python-Solution-Beats-99-or-O(N) | class Solution:
def maxProfit(self, prices: List[int]) -> int:
cumulativeProfit = 0
for i in range(1,len(prices)):
if prices[i-1] < prices[i]:
cumulativeProfit+=(prices[i]-prices[i-1])
return cumulativeProfit | best-time-to-buy-and-sell-stock-ii | Easy Understandable Python Solution Beats 99% | O(N) | perabjoth | 0 | 1 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,297 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2594213/Message-usage-less-than-97.74 | class Solution:
def maxProfit(self, prices: List[int]) -> int:
profit = 0
for i in range(1,len(prices)):
if prices[i]>prices[i-1]:
profit += prices[i]-prices[i-1]
return profit | best-time-to-buy-and-sell-stock-ii | Message usage less than 97.74% | jayeshvarma | 0 | 31 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,298 |
https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/discuss/2584233/Python-easy-solution | class Solution(object):
def maxProfit(self, prices):
"""
:type prices: List[int]
:rtype: int
"""
pos = 0
profit = 0
start = None
while pos < (len(prices) - 1):
today = prices[pos]
tomorrow = prices[pos + 1]
if today < tomorrow: # tomorrow's more expensive
if start is None:
start = today
else: # tomorrow's cheaper so we need to sell if we hold anything
if start is not None:
profit += (today - start)
start = None # reset today - we're not holding anything
pos += 1
if start is not None:
profit += (prices[-1] - start)
return profit | best-time-to-buy-and-sell-stock-ii | Python easy solution | zakmatt | 0 | 57 | best time to buy and sell stock ii | 122 | 0.634 | Medium | 1,299 |
Subsets and Splits